text
stringlengths 6
13.6M
| id
stringlengths 13
176
| metadata
dict | __index_level_0__
int64 0
1.69k
|
---|---|---|---|
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_api_samples/material/context_menu/editable_text_toolbar_builder.2.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('showing and hiding the context menu in TextField with a custom toolbar', (WidgetTester tester) async {
await tester.pumpWidget(
const example.EditableTextToolbarBuilderExampleApp(),
);
expect(BrowserContextMenu.enabled, !kIsWeb);
await tester.tap(find.byType(EditableText));
await tester.pump();
expect(find.byType(AdaptiveTextSelectionToolbar), findsNothing);
// Long pressing the field shows the custom context menu.
await tester.longPress(find.byType(EditableText));
await tester.pumpAndSettle();
expect(find.byType(AdaptiveTextSelectionToolbar), findsNothing);
// The buttons use the default widgets but with custom labels.
switch (defaultTargetPlatform) {
case TargetPlatform.iOS:
expect(find.byType(CupertinoTextSelectionToolbarButton), findsAtLeastNWidgets(1));
case TargetPlatform.android:
case TargetPlatform.fuchsia:
expect(find.byType(TextSelectionToolbarTextButton), findsAtLeastNWidgets(1));
case TargetPlatform.linux:
case TargetPlatform.windows:
expect(find.byType(DesktopTextSelectionToolbarButton), findsAtLeastNWidgets(1));
case TargetPlatform.macOS:
expect(find.byType(CupertinoDesktopTextSelectionToolbarButton), findsAtLeastNWidgets(1));
}
expect(find.text('Copy'), findsNothing);
expect(find.text('Cut'), findsNothing);
expect(find.text('Paste'), findsNothing);
expect(find.text('Select all'), findsNothing);
// Tap to dismiss.
await tester.tapAt(tester.getTopLeft(find.byType(EditableText)));
await tester.pumpAndSettle();
expect(find.byType(AdaptiveTextSelectionToolbar), findsNothing);
expect(find.byType(CupertinoTextSelectionToolbarButton), findsNothing);
expect(find.byType(TextSelectionToolbarTextButton), findsNothing);
expect(find.byType(DesktopTextSelectionToolbarButton), findsNothing);
expect(find.byType(CupertinoDesktopTextSelectionToolbarButton), findsNothing);
expect(find.text('Copy'), findsNothing);
expect(find.text('Cut'), findsNothing);
expect(find.text('Paste'), findsNothing);
expect(find.text('Select all'), findsNothing);
});
}
| flutter/examples/api/test/material/context_menu/editable_text_toolbar_builder.2_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/context_menu/editable_text_toolbar_builder.2_test.dart",
"repo_id": "flutter",
"token_count": 902
} | 612 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/divider/vertical_divider.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Vertical Divider', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: example.VerticalDividerExampleApp(),
),
),
);
expect(find.byType(VerticalDivider), findsOneWidget);
// Divider is positioned horizontally.
Offset expanded = tester.getTopRight(find.byType(Expanded).first);
expect(expanded.dx, tester.getTopLeft(find.byType(VerticalDivider)).dx);
expanded = tester.getTopLeft(find.byType(Expanded).last);
expect(expanded.dx, tester.getTopRight(find.byType(VerticalDivider)).dx);
});
}
| flutter/examples/api/test/material/divider/vertical_divider.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/divider/vertical_divider.0_test.dart",
"repo_id": "flutter",
"token_count": 354
} | 613 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/floating_action_button/floating_action_button.1.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('FloatingActionButton variants', (WidgetTester tester) async {
RawMaterialButton getRawMaterialButtonWidget(Finder finder) {
return tester.widget<RawMaterialButton>(finder);
}
await tester.pumpWidget(
const example.FloatingActionButtonExampleApp(),
);
final ThemeData theme = ThemeData(useMaterial3: true);
expect(find.byType(FloatingActionButton), findsNWidgets(4));
expect(find.byIcon(Icons.add), findsNWidgets(4));
final Finder smallFabMaterialButton = find.byType(RawMaterialButton).at(0);
final RenderBox smallFabRenderBox = tester.renderObject(smallFabMaterialButton);
expect(smallFabRenderBox.size, const Size(48.0, 48.0));
expect(getRawMaterialButtonWidget(smallFabMaterialButton).fillColor, theme.colorScheme.primaryContainer);
expect(getRawMaterialButtonWidget(smallFabMaterialButton).shape, RoundedRectangleBorder(borderRadius: BorderRadius.circular(12.0)));
final Finder regularFABMaterialButton = find.byType(RawMaterialButton).at(1);
final RenderBox regularFABRenderBox = tester.renderObject(regularFABMaterialButton);
expect(regularFABRenderBox.size, const Size(56.0, 56.0));
expect(getRawMaterialButtonWidget(regularFABMaterialButton).fillColor, theme.colorScheme.primaryContainer);
expect(getRawMaterialButtonWidget(regularFABMaterialButton).shape, RoundedRectangleBorder(borderRadius: BorderRadius.circular(16.0)));
final Finder largeFABMaterialButton = find.byType(RawMaterialButton).at(2);
final RenderBox largeFABRenderBox = tester.renderObject(largeFABMaterialButton);
expect(largeFABRenderBox.size, const Size(96.0, 96.0));
expect(getRawMaterialButtonWidget(largeFABMaterialButton).fillColor, theme.colorScheme.primaryContainer);
expect(getRawMaterialButtonWidget(largeFABMaterialButton).shape, RoundedRectangleBorder(borderRadius: BorderRadius.circular(28.0)));
final Finder extendedFABMaterialButton = find.byType(RawMaterialButton).at(3);
final RenderBox extendedFABRenderBox = tester.renderObject(extendedFABMaterialButton);
expect(extendedFABRenderBox.size, within(distance: 0.01, from: const Size(110.3, 56.0)));
expect(getRawMaterialButtonWidget(extendedFABMaterialButton).fillColor, theme.colorScheme.primaryContainer);
expect(getRawMaterialButtonWidget(extendedFABMaterialButton).shape, RoundedRectangleBorder(borderRadius: BorderRadius.circular(16.0)));
});
}
| flutter/examples/api/test/material/floating_action_button/floating_action_button.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/floating_action_button/floating_action_button.1_test.dart",
"repo_id": "flutter",
"token_count": 887
} | 614 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/list_tile/custom_list_item.1.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Custom list item uses AspectRatio and Expanded widgets for the layout', (WidgetTester tester) async {
await tester.pumpWidget(
const example.CustomListItemApp(),
);
// The AspectRatio widget is used to constrain the size of the thumbnail.
AspectRatio thumbnailAspectRatio = tester.widget(find.ancestor(
of: find.byType(Container).first,
matching: find.byType(AspectRatio),
));
expect(thumbnailAspectRatio.aspectRatio, 1.0);
// The Expanded widget is used to control the size of the text.
final Expanded textExpanded = tester.widget(find.ancestor(
of: find.text('Flutter 1.0 Launch'),
matching: find.byType(Expanded).at(0),
));
expect(textExpanded.flex, 1);
// The AspectRatio widget is used to constrain the size of the thumbnail.
thumbnailAspectRatio = tester.widget(find.ancestor(
of: find.byType(Container).last,
matching: find.byType(AspectRatio),
));
expect(thumbnailAspectRatio.aspectRatio, 1.0);
});
}
| flutter/examples/api/test/material/list_tile/custom_list_item.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/list_tile/custom_list_item.1_test.dart",
"repo_id": "flutter",
"token_count": 493
} | 615 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/navigation_drawer/navigation_drawer.0.dart'
as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Navigation bar updates destination on tap',
(WidgetTester tester) async {
await tester.pumpWidget(
const example.NavigationDrawerApp(),
);
await tester.tap(find.text('Open Drawer'));
await tester.pumpAndSettle();
final NavigationDrawer navigationDrawerWidget = tester.firstWidget(find.byType(NavigationDrawer));
/// NavigationDestinations must be rendered
expect(find.text('Messages'), findsNWidgets(2));
expect(find.text('Profile'), findsNWidgets(2));
expect(find.text('Settings'), findsNWidgets(2));
/// Initial index must be zero
expect(navigationDrawerWidget.selectedIndex, 0);
expect(find.text('Page Index = 0'), findsOneWidget);
/// Switch to second tab
await tester.tap(find.ancestor(of: find.text('Profile'), matching: find.byType(InkWell)));
await tester.pumpAndSettle();
expect(find.text('Page Index = 1'), findsOneWidget);
/// Switch to fourth tab
await tester.tap(find.ancestor(of: find.text('Settings'), matching: find.byType(InkWell)));
await tester.pumpAndSettle();
expect(find.text('Page Index = 2'), findsOneWidget);
});
}
| flutter/examples/api/test/material/navigation_drawer/navigation_drawer.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/navigation_drawer/navigation_drawer.0_test.dart",
"repo_id": "flutter",
"token_count": 518
} | 616 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/radio_list_tile/custom_labeled_radio.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('LinkedLabelRadio contains RichText and Radio', (WidgetTester tester) async {
await tester.pumpWidget(
const example.LabeledRadioApp(),
);
// Label text is in a RichText widget with the correct text.
final RichText richText = tester.widget(find.byType(RichText).first);
expect(richText.text.toPlainText(), 'First tappable label text');
// First Radio is initially unchecked.
Radio<bool> radio = tester.widget(find.byType(Radio<bool>).first);
expect(radio.value, true);
expect(radio.groupValue, false);
// Last Radio is initially checked.
radio = tester.widget(find.byType(Radio<bool>).last);
expect(radio.value, false);
expect(radio.groupValue, false);
// Tap the first radio.
await tester.tap(find.byType(Radio<bool>).first);
await tester.pump();
// First Radio is now checked.
radio = tester.widget(find.byType(Radio<bool>).first);
expect(radio.value, true);
expect(radio.groupValue, true);
// Last Radio is now unchecked.
radio = tester.widget(find.byType(Radio<bool>).last);
expect(radio.value, false);
expect(radio.groupValue, true);
});
}
| flutter/examples/api/test/material/radio_list_tile/custom_labeled_radio.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/radio_list_tile/custom_labeled_radio.0_test.dart",
"repo_id": "flutter",
"token_count": 527
} | 617 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/search_anchor/search_anchor.4.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('can search and find options after waiting for fake network delay and debounce delay', (WidgetTester tester) async {
await tester.pumpWidget(const example.SearchAnchorAsyncExampleApp());
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
expect(find.widgetWithText(ListTile, 'aardvark'), findsNothing);
expect(find.widgetWithText(ListTile, 'bobcat'), findsNothing);
expect(find.widgetWithText(ListTile, 'chameleon'), findsNothing);
await tester.enterText(find.byType(SearchBar), 'a');
await tester.pump(example.fakeAPIDuration);
// No results yet, need to also wait for the debounce duration.
expect(find.widgetWithText(ListTile, 'aardvark'), findsNothing);
expect(find.widgetWithText(ListTile, 'bobcat'), findsNothing);
expect(find.widgetWithText(ListTile, 'chameleon'), findsNothing);
await tester.pump(example.debounceDuration);
expect(find.widgetWithText(ListTile, 'aardvark'), findsOneWidget);
expect(find.widgetWithText(ListTile, 'bobcat'), findsOneWidget);
expect(find.widgetWithText(ListTile, 'chameleon'), findsOneWidget);
await tester.enterText(find.byType(SearchBar), 'aa');
await tester.pump(example.debounceDuration + example.fakeAPIDuration);
expect(find.widgetWithText(ListTile, 'aardvark'), findsOneWidget);
expect(find.widgetWithText(ListTile, 'bobcat'), findsNothing);
expect(find.widgetWithText(ListTile, 'chameleon'), findsNothing);
});
testWidgets('debounce is reset each time a character is entered', (WidgetTester tester) async {
await tester.pumpWidget(const example.SearchAnchorAsyncExampleApp());
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
await tester.enterText(find.byType(SearchBar), 'c');
await tester.pump(example.debounceDuration - const Duration(milliseconds: 100));
expect(find.widgetWithText(ListTile, 'aardvark'), findsNothing);
expect(find.widgetWithText(ListTile, 'bobcat'), findsNothing);
expect(find.widgetWithText(ListTile, 'chameleon'), findsNothing);
await tester.enterText(find.byType(SearchBar), 'ch');
await tester.pump(example.debounceDuration - const Duration(milliseconds: 100));
expect(find.widgetWithText(ListTile, 'aardvark'), findsNothing);
expect(find.widgetWithText(ListTile, 'bobcat'), findsNothing);
expect(find.widgetWithText(ListTile, 'chameleon'), findsNothing);
await tester.enterText(find.byType(SearchBar), 'cha');
await tester.pump(example.debounceDuration - const Duration(milliseconds: 100));
expect(find.widgetWithText(ListTile, 'aardvark'), findsNothing);
expect(find.widgetWithText(ListTile, 'bobcat'), findsNothing);
expect(find.widgetWithText(ListTile, 'chameleon'), findsNothing);
await tester.enterText(find.byType(SearchBar), 'cham');
await tester.pump(example.debounceDuration - const Duration(milliseconds: 100));
// Despite the total elapsed time being greater than debounceDuration +
// fakeAPIDuration, the search has not yet completed, because the debounce
// was reset each time text input happened.
expect(find.widgetWithText(ListTile, 'aardvark'), findsNothing);
expect(find.widgetWithText(ListTile, 'bobcat'), findsNothing);
expect(find.widgetWithText(ListTile, 'chameleon'), findsNothing);
await tester.enterText(find.byType(SearchBar), 'chame');
await tester.pump(example.debounceDuration + example.fakeAPIDuration);
expect(find.widgetWithText(ListTile, 'aardvark'), findsNothing);
expect(find.widgetWithText(ListTile, 'bobcat'), findsNothing);
expect(find.widgetWithText(ListTile, 'chameleon'), findsOneWidget);
});
}
| flutter/examples/api/test/material/search_anchor/search_anchor.4_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/search_anchor/search_anchor.4_test.dart",
"repo_id": "flutter",
"token_count": 1340
} | 618 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/material/switch_list_tile/switch_list_tile.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('SwitchListTile can be toggled', (WidgetTester tester) async {
await tester.pumpWidget(
const example.SwitchListTileApp(),
);
expect(find.byType(SwitchListTile), findsOneWidget);
SwitchListTile switchListTile = tester.widget(find.byType(SwitchListTile));
expect(switchListTile.value, isFalse);
await tester.tap(find.byType(SwitchListTile));
await tester.pumpAndSettle();
switchListTile = tester.widget(find.byType(SwitchListTile));
expect(switchListTile.value, isTrue);
});
}
| flutter/examples/api/test/material/switch_list_tile/switch_list_tile.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/material/switch_list_tile/switch_list_tile.0_test.dart",
"repo_id": "flutter",
"token_count": 304
} | 619 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_api_samples/painting/axis_direction/axis_direction.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Example app has radio buttons to toggle AxisDirection', (WidgetTester tester) async {
await tester.pumpWidget(
const example.ExampleApp(),
);
expect(find.byType(Radio<AxisDirection>), findsNWidgets(4));
final RenderViewport viewport = tester.renderObject(find.byType(Viewport));
expect(find.text('AxisDirection.down'), findsOneWidget);
expect(find.text('Axis.vertical'), findsOneWidget);
expect(find.text('GrowthDirection.forward'), findsOneWidget);
expect(find.byIcon(Icons.arrow_downward_rounded), findsNWidgets(2));
expect(viewport.axisDirection, AxisDirection.down);
await tester.tap(
find.byWidgetPredicate((Widget widget) {
return widget is Radio<AxisDirection> && widget.value == AxisDirection.up;
})
);
await tester.pumpAndSettle();
expect(find.text('AxisDirection.up'), findsOneWidget);
expect(find.text('Axis.vertical'), findsOneWidget);
expect(find.text('GrowthDirection.forward'), findsOneWidget);
expect(find.byIcon(Icons.arrow_upward_rounded), findsNWidgets(2));
expect(viewport.axisDirection, AxisDirection.up);
});
}
| flutter/examples/api/test/painting/axis_direction.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/painting/axis_direction.0_test.dart",
"repo_id": "flutter",
"token_count": 537
} | 620 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_api_samples/services/system_chrome/system_chrome.set_system_u_i_overlay_style.1.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('AnnotatedRegion can change system overlays style.', (WidgetTester tester) async {
await tester.pumpWidget(
const example.SystemOverlayStyleApp(),
);
final SystemUiOverlayStyle? firstStyle = SystemChrome.latestStyle;
await tester.tap(find.byType(ElevatedButton));
await tester.pump();
final SystemUiOverlayStyle? secondStyle = SystemChrome.latestStyle;
expect(secondStyle?.statusBarColor, isNot(firstStyle?.statusBarColor));
expect(secondStyle?.systemNavigationBarColor, isNot(firstStyle?.systemNavigationBarColor));
await tester.tap(find.byType(ElevatedButton));
await tester.pump();
final SystemUiOverlayStyle? thirdStyle = SystemChrome.latestStyle;
expect(thirdStyle?.statusBarColor, isNot(secondStyle?.statusBarColor));
expect(thirdStyle?.systemNavigationBarColor, isNot(secondStyle?.systemNavigationBarColor));
});
}
| flutter/examples/api/test/services/system_chrome/system_chrome.set_system_u_i_overlay_style.1_test.dart/0 | {
"file_path": "flutter/examples/api/test/services/system_chrome/system_chrome.set_system_u_i_overlay_style.1_test.dart",
"repo_id": "flutter",
"token_count": 437
} | 621 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_api_samples/widgets/app/widgets_app.widgets_app.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('WidgetsApp test', (WidgetTester tester) async {
await tester.pumpWidget(
const example.WidgetsAppExampleApp(),
);
expect(find.text('Hello World'), findsOneWidget);
});
}
| flutter/examples/api/test/widgets/app/widgets_app.widgets_app.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/app/widgets_app.widgets_app.0_test.dart",
"repo_id": "flutter",
"token_count": 179
} | 622 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/basic/indexed_stack.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('has correct forward rendering mechanism', (WidgetTester tester) async {
await tester.pumpWidget(const example.IndexedStackApp());
final Finder gesture2 = find.byKey(const Key('gesture2'));
final Element containerFinder = find.byKey(const Key('Dash'), skipOffstage: false).evaluate().first;
expect(containerFinder.renderObject!.debugNeedsPaint, false);
final Element containerFinder1 = find.byKey(const Key('John'), skipOffstage: false).evaluate().first;
expect(containerFinder1.renderObject!.debugNeedsPaint, true);
final Element containerFinder2 = find.byKey(const Key('Mary'), skipOffstage: false).evaluate().first;
expect(containerFinder2.renderObject!.debugNeedsPaint, true);
await tester.tap(gesture2);
await tester.pump();
expect(containerFinder.renderObject!.debugNeedsPaint, false);
expect(containerFinder1.renderObject!.debugNeedsPaint, false);
expect(containerFinder2.renderObject!.debugNeedsPaint, true);
await tester.tap(gesture2);
await tester.pump();
expect(containerFinder.renderObject!.debugNeedsPaint, false);
expect(containerFinder1.renderObject!.debugNeedsPaint, false);
expect(containerFinder2.renderObject!.debugNeedsPaint, false);
});
testWidgets('has correct backward rendering mechanism', (WidgetTester tester) async {
await tester.pumpWidget(const example.IndexedStackApp());
final Finder gesture1 = find.byKey(const Key('gesture1'));
final Element containerFinder = find.byKey(const Key('Dash'), skipOffstage: false).evaluate().first;
final Element containerFinder1 = find.byKey(const Key('John'), skipOffstage: false).evaluate().first;
final Element containerFinder2 = find.byKey(const Key('Mary'), skipOffstage: false).evaluate().first;
await tester.tap(gesture1);
await tester.pump();
expect(containerFinder.renderObject!.debugNeedsPaint, false);
expect(containerFinder1.renderObject!.debugNeedsPaint, true);
expect(containerFinder2.renderObject!.debugNeedsPaint, false);
await tester.tap(gesture1);
await tester.pump();
expect(containerFinder.renderObject!.debugNeedsPaint, false);
expect(containerFinder1.renderObject!.debugNeedsPaint, false);
expect(containerFinder2.renderObject!.debugNeedsPaint, false);
});
testWidgets('has correct element addition handling', (WidgetTester tester) async {
await tester.pumpWidget(const example.IndexedStackApp());
expect(find.byType(example.PersonTracker), findsOneWidget);
expect(find.byType(example.PersonTracker, skipOffstage: false), findsNWidgets(3));
final Finder textField = find.byType(TextField);
await tester.enterText(textField, 'hello');
await tester.testTextInput.receiveAction(TextInputAction.done);
await tester.pump();
expect(find.byType(example.PersonTracker, skipOffstage: false), findsNWidgets(4));
await tester.enterText(textField, 'hello1');
await tester.testTextInput.receiveAction(TextInputAction.done);
await tester.pump();
expect(find.byType(example.PersonTracker, skipOffstage: false), findsNWidgets(5));
});
testWidgets('has state preservation', (WidgetTester tester) async {
await tester.pumpWidget(const example.IndexedStackApp());
final Finder gesture1 = find.byKey(const Key('gesture1'));
final Finder gesture2 = find.byKey(const Key('gesture2'));
final Finder containerFinder = find.byKey(const Key('Dash'));
final Finder incrementFinder = find.byKey(const Key('incrementDash'));
Finder counterFinder(int score) {
return find.descendant(of: containerFinder, matching: find.text('Score: $score'));
}
expect(counterFinder(0), findsOneWidget);
await tester.tap(incrementFinder);
await tester.pump();
expect(counterFinder(1), findsOneWidget);
await tester.tap(gesture2);
await tester.pump();
await tester.tap(gesture1);
await tester.pump();
expect(counterFinder(1), findsOneWidget);
expect(counterFinder(0), findsNothing);
});
}
| flutter/examples/api/test/widgets/basic/indexed_stack.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/basic/indexed_stack.0_test.dart",
"repo_id": "flutter",
"token_count": 1443
} | 623 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_api_samples/widgets/heroes/hero.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Has Hero animation', (WidgetTester tester) async {
await tester.pumpWidget(
const example.HeroApp(),
);
expect(find.text('Hero Sample'), findsOneWidget);
await tester.tap(find.byType(Container));
await tester.pump();
Size heroSize = tester.getSize(find.byType(Container));
// Jump 25% into the transition (total length = 300ms)
await tester.pump(const Duration(milliseconds: 75)); // 25% of 300ms
heroSize = tester.getSize(find.byType(Container));
expect(heroSize.width.roundToDouble(), 103.0);
expect(heroSize.height.roundToDouble(), 60.0);
// Jump to 50% into the transition.
await tester.pump(const Duration(milliseconds: 75)); // 25% of 300ms
heroSize = tester.getSize(find.byType(Container));
expect(heroSize.width.roundToDouble(), 189.0);
expect(heroSize.height.roundToDouble(), 146.0);
// Jump to 75% into the transition.
await tester.pump(const Duration(milliseconds: 75)); // 25% of 300ms
heroSize = tester.getSize(find.byType(Container));
expect(heroSize.width.roundToDouble(), 199.0);
expect(heroSize.height.roundToDouble(), 190.0);
// Jump to 100% into the transition.
await tester.pump(const Duration(milliseconds: 75)); // 25% of 300ms
heroSize = tester.getSize(find.byType(Container));
expect(heroSize, const Size(200.0, 200.0));
expect(find.byIcon(Icons.arrow_back), findsOneWidget);
await tester.tap(find.byIcon(Icons.arrow_back));
await tester.pump();
// Jump 25% into the transition (total length = 300ms)
await tester.pump(const Duration(milliseconds: 75)); // 25% of 300ms
heroSize = tester.getSize(find.byType(Container));
expect(heroSize.width.roundToDouble(), 199.0);
expect(heroSize.height.roundToDouble(), 190.0);
// Jump to 50% into the transition.
await tester.pump(const Duration(milliseconds: 75)); // 25% of 300ms
heroSize = tester.getSize(find.byType(Container));
expect(heroSize.width.roundToDouble(), 189.0);
expect(heroSize.height.roundToDouble(), 146.0);
// Jump to 75% into the transition.
await tester.pump(const Duration(milliseconds: 75)); // 25% of 300ms
heroSize = tester.getSize(find.byType(Container));
expect(heroSize.width.roundToDouble(), 103.0);
expect(heroSize.height.roundToDouble(), 60.0);
// Jump to 100% into the transition.
await tester.pump(const Duration(milliseconds: 75)); // 25% of 300ms
heroSize = tester.getSize(find.byType(Container));
expect(heroSize, const Size(50.0, 50.0));
});
}
| flutter/examples/api/test/widgets/heroes/hero.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/heroes/hero.0_test.dart",
"repo_id": "flutter",
"token_count": 1033
} | 624 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_api_samples/widgets/routes/route_observer.0.dart' as example;
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('RouteObserver notifies RouteAware widget', (WidgetTester tester) async {
await tester.pumpWidget(
const example.RouteObserverApp(),
);
// Check the initial RouteObserver logs.
expect(find.text('didPush'), findsOneWidget);
// Tap on the button to push a new route.
await tester.tap(find.text('Go to next page'));
await tester.pumpAndSettle();
// Tap on the button to go back to the previous route.
await tester.tap(find.text('Go back to RouteAware page'));
await tester.pumpAndSettle();
// Check the RouteObserver logs after the route is popped.
expect(find.text('didPush'), findsOneWidget);
expect(find.text('didPopNext'), findsOneWidget);
// Tap on the button to push a new route again.
await tester.tap(find.text('Go to next page'));
await tester.pumpAndSettle();
// Tap on the button to go back to the previous route again.
await tester.tap(find.text('Go back to RouteAware page'));
await tester.pumpAndSettle();
// Check the RouteObserver logs after the route is popped again.
expect(find.text('didPush'), findsOneWidget);
expect(find.text('didPopNext'), findsNWidgets(2));
});
}
| flutter/examples/api/test/widgets/routes/route_observer.0_test.dart/0 | {
"file_path": "flutter/examples/api/test/widgets/routes/route_observer.0_test.dart",
"repo_id": "flutter",
"token_count": 507
} | 625 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#import <UIKit/UIKit.h>
@protocol NativeViewControllerDelegate <NSObject>
- (void)didTapIncrementButton;
@end
@interface NativeViewController: UIViewController
@property (strong, nonatomic) id<NativeViewControllerDelegate> delegate;
- (void) didReceiveIncrement;
@end
| flutter/examples/flutter_view/ios/Runner/NativeViewController.h/0 | {
"file_path": "flutter/examples/flutter_view/ios/Runner/NativeViewController.h",
"repo_id": "flutter",
"token_count": 129
} | 626 |
#include "../../Flutter/Flutter-Debug.xcconfig"
#include "Warnings.xcconfig"
| flutter/examples/flutter_view/macos/Runner/Configs/Debug.xcconfig/0 | {
"file_path": "flutter/examples/flutter_view/macos/Runner/Configs/Debug.xcconfig",
"repo_id": "flutter",
"token_count": 32
} | 627 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// Contents of this file should be generated automatically by
// dev/tools/bin/generate_gradle_lockfiles.dart, but currently are not.
// See #141540.
allprojects {
repositories {
google()
mavenCentral()
}
}
rootProject.buildDir = file("../build")
subprojects {
project.buildDir = file("${rootProject.buildDir}/${project.name}")
}
subprojects {
project.evaluationDependsOn(":app")
dependencyLocking {
ignoredDependencies.add("io.flutter:*")
lockFile = file("${rootProject.projectDir}/project-${project.name}.lockfile")
if (!project.hasProperty("local-engine-repo")) {
lockAllConfigurations()
}
}
}
tasks.register<Delete>("clean") {
delete(rootProject.buildDir)
}
| flutter/examples/hello_world/android/build.gradle.kts/0 | {
"file_path": "flutter/examples/hello_world/android/build.gradle.kts",
"repo_id": "flutter",
"token_count": 334
} | 628 |
#include "../../Flutter/Flutter-Release.xcconfig"
#include "Warnings.xcconfig"
| flutter/examples/image_list/macos/Runner/Configs/Release.xcconfig/0 | {
"file_path": "flutter/examples/image_list/macos/Runner/Configs/Release.xcconfig",
"repo_id": "flutter",
"token_count": 32
} | 629 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This example shows how to perform a simple animation using the raw interface
// to the engine.
import 'dart:math' as math;
import 'dart:typed_data';
import 'dart:ui' as ui;
// The FlutterView into which this example will draw; set in the main method.
late final ui.FlutterView view;
void beginFrame(Duration timeStamp) {
// The timeStamp argument to beginFrame indicates the timing information we
// should use to clock our animations. It's important to use timeStamp rather
// than reading the system time because we want all the parts of the system to
// coordinate the timings of their animations. If each component read the
// system clock independently, the animations that we processed later would be
// slightly ahead of the animations we processed earlier.
// PAINT
final ui.Rect paintBounds = ui.Offset.zero & (view.physicalSize / view.devicePixelRatio);
final ui.PictureRecorder recorder = ui.PictureRecorder();
final ui.Canvas canvas = ui.Canvas(recorder, paintBounds);
canvas.translate(paintBounds.width / 2.0, paintBounds.height / 2.0);
// Here we determine the rotation according to the timeStamp given to us by
// the engine.
final double t = timeStamp.inMicroseconds / Duration.microsecondsPerMillisecond / 1800.0;
canvas.rotate(math.pi * (t % 1.0));
canvas.drawRect(
const ui.Rect.fromLTRB(-100.0, -100.0, 100.0, 100.0),
ui.Paint()..color = const ui.Color.fromARGB(255, 0, 255, 0),
);
final ui.Picture picture = recorder.endRecording();
// COMPOSITE
final double devicePixelRatio = view.devicePixelRatio;
final Float64List deviceTransform = Float64List(16)
..[0] = devicePixelRatio
..[5] = devicePixelRatio
..[10] = 1.0
..[15] = 1.0;
final ui.SceneBuilder sceneBuilder = ui.SceneBuilder()
..pushTransform(deviceTransform)
..addPicture(ui.Offset.zero, picture)
..pop();
view.render(sceneBuilder.build());
// After rendering the current frame of the animation, we ask the engine to
// schedule another frame. The engine will call beginFrame again when its time
// to produce the next frame.
ui.PlatformDispatcher.instance.scheduleFrame();
}
void main() {
// TODO(goderbauer): Create a window if embedder doesn't provide an implicit view to draw into.
assert(ui.PlatformDispatcher.instance.implicitView != null);
view = ui.PlatformDispatcher.instance.implicitView!;
ui.PlatformDispatcher.instance
..onBeginFrame = beginFrame
..scheduleFrame();
}
| flutter/examples/layers/raw/spinning_square.dart/0 | {
"file_path": "flutter/examples/layers/raw/spinning_square.dart",
"repo_id": "flutter",
"token_count": 814
} | 630 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter_test/flutter_test.dart';
import '../rendering/src/sector_layout.dart';
void main() {
group('hit testing', () {
test('SectorHitTestResult wrapping HitTestResult', () {
final HitTestEntry entry1 = HitTestEntry(_DummyHitTestTarget());
final HitTestEntry entry2 = HitTestEntry(_DummyHitTestTarget());
final HitTestEntry entry3 = HitTestEntry(_DummyHitTestTarget());
final HitTestResult wrapped = HitTestResult();
wrapped.add(entry1);
expect(wrapped.path, equals(<HitTestEntry>[entry1]));
final SectorHitTestResult wrapping = SectorHitTestResult.wrap(wrapped);
expect(wrapping.path, equals(<HitTestEntry>[entry1]));
expect(wrapping.path, same(wrapped.path));
wrapping.add(entry2);
expect(wrapping.path, equals(<HitTestEntry>[entry1, entry2]));
expect(wrapped.path, equals(<HitTestEntry>[entry1, entry2]));
wrapped.add(entry3);
expect(wrapping.path, equals(<HitTestEntry>[entry1, entry2, entry3]));
expect(wrapped.path, equals(<HitTestEntry>[entry1, entry2, entry3]));
});
});
}
class _DummyHitTestTarget implements HitTestTarget {
@override
void handleEvent(PointerEvent event, HitTestEntry entry) {
// Nothing to do.
}
}
| flutter/examples/layers/test/sector_layout_test.dart/0 | {
"file_path": "flutter/examples/layers/test/sector_layout_test.dart",
"repo_id": "flutter",
"token_count": 513
} | 631 |
#include "ephemeral/Flutter-Generated.xcconfig"
| flutter/examples/platform_channel/macos/Flutter/Flutter-Debug.xcconfig/0 | {
"file_path": "flutter/examples/platform_channel/macos/Flutter/Flutter-Debug.xcconfig",
"repo_id": "flutter",
"token_count": 19
} | 632 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import Cocoa
import FlutterMacOS
class MainFlutterWindow: NSWindow {
private let powerSource = PowerSource()
private let stateChangeHandler = PowerSourceStateChangeHandler()
private var eventSink: FlutterEventSink?
override func awakeFromNib() {
let flutterViewController = FlutterViewController()
let windowFrame = self.frame
self.contentViewController = flutterViewController
self.displayIfNeeded()
self.setFrame(windowFrame, display: true)
// Register battery method channel.
let registrar = flutterViewController.registrar(forPlugin: "BatteryLevel")
let batteryChannel = FlutterMethodChannel(
name: "samples.flutter.io/battery",
binaryMessenger: registrar.messenger)
batteryChannel.setMethodCallHandler { [powerSource = self.powerSource] (call, result) in
switch call.method {
case "getBatteryLevel":
guard powerSource.hasBattery() else {
result(
FlutterError(
code: "NO_BATTERY",
message: "Device does not have a battery",
details: nil))
return
}
guard let level = powerSource.getCurrentCapacity() else {
result(
FlutterError(
code: "UNAVAILABLE",
message: "Battery info unavailable",
details: nil))
return
}
result(level)
default:
result(FlutterMethodNotImplemented)
}
}
// Register charging event channel.
let chargingChannel = FlutterEventChannel(
name: "samples.flutter.io/charging",
binaryMessenger: registrar.messenger)
chargingChannel.setStreamHandler(self)
RegisterGeneratedPlugins(registry: flutterViewController)
super.awakeFromNib()
}
/// Emit a power status event to the registered event sink.
func emitPowerStatusEvent() {
if let sink = self.eventSink {
switch self.powerSource.getPowerState() {
case .ac:
sink("charging")
case .battery:
sink("discharging")
case .unknown:
sink("UNAVAILABLE")
}
}
}
}
extension MainFlutterWindow: FlutterStreamHandler {
func onListen(withArguments arguments: Any?, eventSink events: @escaping FlutterEventSink)
-> FlutterError?
{
self.eventSink = events
self.emitPowerStatusEvent()
self.stateChangeHandler.delegate = self
return nil
}
func onCancel(withArguments arguments: Any?) -> FlutterError? {
self.stateChangeHandler.delegate = nil
self.eventSink = nil
return nil
}
}
extension MainFlutterWindow: PowerSourceStateChangeDelegate {
func didChangePowerSourceState() {
self.emitPowerStatusEvent()
}
}
| flutter/examples/platform_channel/macos/Runner/MainFlutterWindow.swift/0 | {
"file_path": "flutter/examples/platform_channel/macos/Runner/MainFlutterWindow.swift",
"repo_id": "flutter",
"token_count": 1069
} | 633 |
#include "Generated.xcconfig"
| flutter/examples/platform_view/ios/Flutter/Release.xcconfig/0 | {
"file_path": "flutter/examples/platform_view/ios/Flutter/Release.xcconfig",
"repo_id": "flutter",
"token_count": 12
} | 634 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
void main() {
runApp(const PlatformView());
}
class PlatformView extends StatelessWidget {
const PlatformView({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Platform View',
theme: ThemeData(
primarySwatch: Colors.grey,
),
home: const MyHomePage(title: 'Platform View'),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key, required this.title});
final String title;
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
static const MethodChannel _methodChannel =
MethodChannel('samples.flutter.io/platform_view');
int _counter = 0;
void _incrementCounter() {
setState(() {
_counter++;
});
}
static Widget get _buttonText {
return switch (defaultTargetPlatform) {
TargetPlatform.android => const Text('Continue in Android view'),
TargetPlatform.iOS => const Text('Continue in iOS view'),
TargetPlatform.windows => const Text('Continue in Windows view'),
TargetPlatform.macOS => const Text('Continue in macOS view'),
TargetPlatform.linux => const Text('Continue in Linux view'),
TargetPlatform.fuchsia => throw UnimplementedError('Platform not yet implemented'),
};
}
Future<void> _launchPlatformCount() async {
final int? platformCounter =
await _methodChannel.invokeMethod('switchView', _counter);
setState(() {
_counter = platformCounter!;
});
}
@override
Widget build(BuildContext context) => Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Expanded(
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'Button tapped $_counter time${ _counter == 1 ? '' : 's' }.',
style: const TextStyle(fontSize: 17.0),
),
Padding(
padding: const EdgeInsets.all(18.0),
child: ElevatedButton(
onPressed: _launchPlatformCount,
child: _buttonText,
),
),
],
),
),
),
Container(
padding: const EdgeInsets.only(bottom: 15.0, left: 5.0),
child: Row(
children: <Widget>[
Image.asset('assets/flutter-mark-square-64.png', scale: 1.5),
const Text(
'Flutter',
style: TextStyle(fontSize: 30.0),
),
],
),
),
],
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: const Icon(Icons.add),
),
);
}
| flutter/examples/platform_view/lib/main.dart/0 | {
"file_path": "flutter/examples/platform_view/lib/main.dart",
"repo_id": "flutter",
"token_count": 1585
} | 635 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include "flutter_window.h"
#include <WinUser.h>
#include <flutter/flutter_engine.h>
#include <flutter/method_channel.h>
#include <flutter/plugin_registrar_windows.h>
#include <flutter/standard_method_codec.h>
#include <cstdint>
#include <memory>
#include <optional>
#include <string>
#include "flutter/generated_plugin_registrant.h"
void RegisterMethodChannel(flutter::FlutterEngine* engine) {
FlutterDesktopPluginRegistrarRef plugin_registrar_ref =
engine->GetRegistrarForPlugin("platform_view");
flutter::PluginRegistrarWindows* registrar =
flutter::PluginRegistrarManager::GetInstance()
->GetRegistrar<flutter::PluginRegistrarWindows>(plugin_registrar_ref);
auto channel =
std::make_unique<flutter::MethodChannel<flutter::EncodableValue>>(
registrar->messenger(), "samples.flutter.io/platform_view",
&flutter::StandardMethodCodec::GetInstance());
channel->SetMethodCallHandler([](const auto& call, auto result) {
int counter = std::get<int32_t>(*call.arguments());
std::string msg = std::string("Pressed the button ") +
std::to_string(counter) + " times!";
MessageBoxA(NULL, msg.c_str(), "Popup", MB_OK);
result->Success(flutter::EncodableValue(counter));
});
}
FlutterWindow::FlutterWindow(const flutter::DartProject& project)
: project_(project) {}
FlutterWindow::~FlutterWindow() {}
bool FlutterWindow::OnCreate() {
if (!Win32Window::OnCreate()) {
return false;
}
RECT frame = GetClientArea();
// The size here must match the window dimensions to avoid unnecessary surface
// creation / destruction in the startup path.
flutter_controller_ = std::make_unique<flutter::FlutterViewController>(
frame.right - frame.left, frame.bottom - frame.top, project_);
// Ensure that basic setup of the controller was successful.
if (!flutter_controller_->engine() || !flutter_controller_->view()) {
return false;
}
RegisterPlugins(flutter_controller_->engine());
RegisterMethodChannel(flutter_controller_->engine());
SetChildContent(flutter_controller_->view()->GetNativeWindow());
return true;
}
void FlutterWindow::OnDestroy() {
if (flutter_controller_) {
flutter_controller_ = nullptr;
}
Win32Window::OnDestroy();
}
LRESULT
FlutterWindow::MessageHandler(HWND hwnd, UINT const message,
WPARAM const wparam,
LPARAM const lparam) noexcept {
// Give Flutter, including plugins, an opportunity to handle window messages.
if (flutter_controller_) {
std::optional<LRESULT> result =
flutter_controller_->HandleTopLevelWindowProc(hwnd, message, wparam,
lparam);
if (result) {
return *result;
}
}
switch (message) {
case WM_FONTCHANGE:
flutter_controller_->engine()->ReloadSystemFonts();
break;
}
return Win32Window::MessageHandler(hwnd, message, wparam, lparam);
}
| flutter/examples/platform_view/windows/runner/flutter_window.cpp/0 | {
"file_path": "flutter/examples/platform_view/windows/runner/flutter_window.cpp",
"repo_id": "flutter",
"token_count": 1151
} | 636 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
/// Flutter widgets implementing the current iOS design language.
///
/// To use, import `package:flutter/cupertino.dart`.
///
/// This library is designed for apps that run on iOS. For apps that may also
/// run on other operating systems, we encourage use of other widgets, for
/// example the [Material
/// Design](https://flutter.dev/docs/development/ui/widgets/material) set.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=3PdUaidHc-E}
///
/// See also:
///
/// * [flutter.dev/widgets/cupertino](https://flutter.dev/docs/development/ui/widgets/cupertino)
/// for a catalog of all Cupertino widgets.
/// * [flutter.dev/widgets](https://flutter.dev/widgets/)
/// for a catalog of commonly-used Flutter widgets.
library cupertino;
export 'src/cupertino/activity_indicator.dart';
export 'src/cupertino/adaptive_text_selection_toolbar.dart';
export 'src/cupertino/app.dart';
export 'src/cupertino/bottom_tab_bar.dart';
export 'src/cupertino/button.dart';
export 'src/cupertino/checkbox.dart';
export 'src/cupertino/colors.dart';
export 'src/cupertino/constants.dart';
export 'src/cupertino/context_menu.dart';
export 'src/cupertino/context_menu_action.dart';
export 'src/cupertino/date_picker.dart';
export 'src/cupertino/debug.dart';
export 'src/cupertino/desktop_text_selection.dart';
export 'src/cupertino/desktop_text_selection_toolbar.dart';
export 'src/cupertino/desktop_text_selection_toolbar_button.dart';
export 'src/cupertino/dialog.dart';
export 'src/cupertino/form_row.dart';
export 'src/cupertino/form_section.dart';
export 'src/cupertino/icon_theme_data.dart';
export 'src/cupertino/icons.dart';
export 'src/cupertino/interface_level.dart';
export 'src/cupertino/list_section.dart';
export 'src/cupertino/list_tile.dart';
export 'src/cupertino/localizations.dart';
export 'src/cupertino/magnifier.dart';
export 'src/cupertino/nav_bar.dart';
export 'src/cupertino/page_scaffold.dart';
export 'src/cupertino/picker.dart';
export 'src/cupertino/radio.dart';
export 'src/cupertino/refresh.dart';
export 'src/cupertino/route.dart';
export 'src/cupertino/scrollbar.dart';
export 'src/cupertino/search_field.dart';
export 'src/cupertino/segmented_control.dart';
export 'src/cupertino/slider.dart';
export 'src/cupertino/sliding_segmented_control.dart';
export 'src/cupertino/spell_check_suggestions_toolbar.dart';
export 'src/cupertino/switch.dart';
export 'src/cupertino/tab_scaffold.dart';
export 'src/cupertino/tab_view.dart';
export 'src/cupertino/text_field.dart';
export 'src/cupertino/text_form_field_row.dart';
export 'src/cupertino/text_selection.dart';
export 'src/cupertino/text_selection_toolbar.dart';
export 'src/cupertino/text_selection_toolbar_button.dart';
export 'src/cupertino/text_theme.dart';
export 'src/cupertino/theme.dart';
export 'src/cupertino/thumb_painter.dart';
export 'widgets.dart';
| flutter/packages/flutter/lib/cupertino.dart/0 | {
"file_path": "flutter/packages/flutter/lib/cupertino.dart",
"repo_id": "flutter",
"token_count": 1091
} | 637 |
# Copyright 2014 The Flutter Authors. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
# For details regarding the *Flutter Fix* feature, see
# https://flutter.dev/docs/development/tools/flutter-fix
# Please add new fixes to the top of the file, separated by one blank line
# from other fixes. In a comment, include a link to the PR where the change
# requiring the fix was made.
# Every fix must be tested. See the flutter/packages/flutter/test_fixes/README.md
# file for instructions on testing these data driven fixes.
# For documentation about this file format, see
# https://dart.dev/go/data-driven-fixes.
# * Fixes in this file are for Actions from the Widgets library. *
# For fixes to
# * BuildContext: fix_build_context.yaml
# * Element: fix_element.yaml
# * ListWheelScrollView: fix_list_wheel_scroll_view.yaml
# * Widgets (general): fix_widgets.yaml
version: 1
transforms:
# Changes made in https://github.com/flutter/flutter/pull/68921.
- title: "Migrate from 'nullOk'"
date: 2021-01-27
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'invoke'
inClass: 'Actions'
oneOf:
- if: "nullOk == 'true'"
changes:
- kind: 'rename'
newName: 'maybeInvoke'
- kind: 'removeParameter'
name: 'nullOk'
- if: "nullOk == 'false'"
changes:
- kind: 'removeParameter'
name: 'nullOk'
variables:
nullOk:
kind: 'fragment'
value: 'arguments[nullOk]'
# Changes made in https://github.com/flutter/flutter/pull/68921.
- title: "Migrate from 'nullOk'"
date: 2021-01-27
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'find'
inClass: 'Actions'
oneOf:
- if: "nullOk == 'true'"
changes:
- kind: 'rename'
newName: 'maybeFind'
- kind: 'removeParameter'
name: 'nullOk'
- if: "nullOk == 'false'"
changes:
- kind: 'removeParameter'
name: 'nullOk'
variables:
nullOk:
kind: 'fragment'
value: 'arguments[nullOk]'
# Changes made in https://github.com/flutter/flutter/pull/68921.
- title: "Migrate from 'nullOk'"
date: 2021-01-27
element:
uris: [ 'widgets.dart', 'material.dart', 'cupertino.dart' ]
method: 'handler'
inClass: 'Actions'
changes:
- kind: 'removeParameter'
name: 'nullOk'
# Before adding a new fix: read instructions at the top of this file.
| flutter/packages/flutter/lib/fix_data/fix_widgets/fix_actions.yaml/0 | {
"file_path": "flutter/packages/flutter/lib/fix_data/fix_widgets/fix_actions.yaml",
"repo_id": "flutter",
"token_count": 1100
} | 638 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
/// The Flutter semantics package.
///
/// To use, import `package:flutter/semantics.dart`.
///
/// The [SemanticsEvent] classes define the protocol for sending semantic events
/// to the platform.
///
/// The [SemanticsNode] hierarchy represents the semantic structure of the UI
/// and is used by the platform-specific accessibility services.
library semantics;
export 'dart:ui' show LocaleStringAttribute, SpellOutStringAttribute;
export 'src/semantics/binding.dart';
export 'src/semantics/debug.dart';
export 'src/semantics/semantics.dart';
export 'src/semantics/semantics_event.dart';
export 'src/semantics/semantics_service.dart';
| flutter/packages/flutter/lib/semantics.dart/0 | {
"file_path": "flutter/packages/flutter/lib/semantics.dart",
"repo_id": "flutter",
"token_count": 222
} | 639 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show Brightness, Color;
import '../../foundation.dart';
import '../widgets/basic.dart';
import '../widgets/framework.dart';
import '../widgets/media_query.dart';
import 'interface_level.dart';
import 'theme.dart';
// Examples can assume:
// late Widget child;
// late BuildContext context;
/// A palette of [Color] constants that describe colors commonly used when
/// matching the iOS platform aesthetics.
///
/// ## Color palettes
///
/// ### Basic Colors
/// 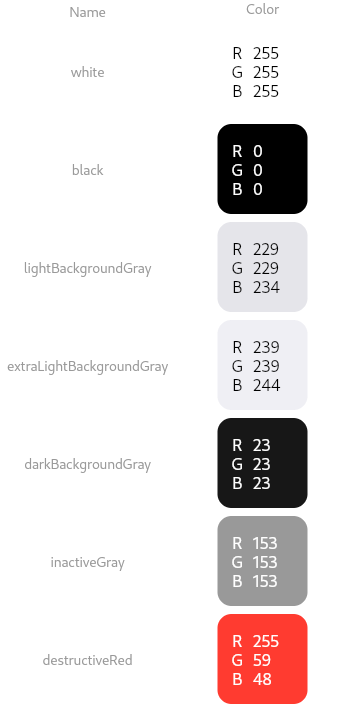
///
/// ### Active Colors
/// 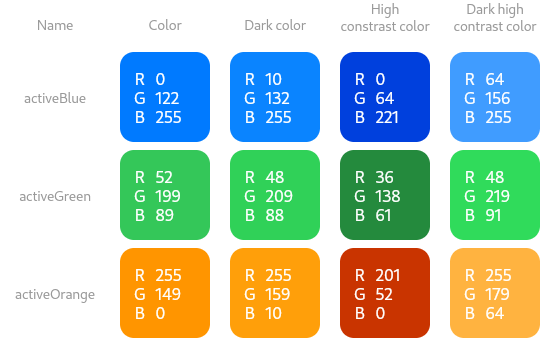
///
/// ### System Colors
/// 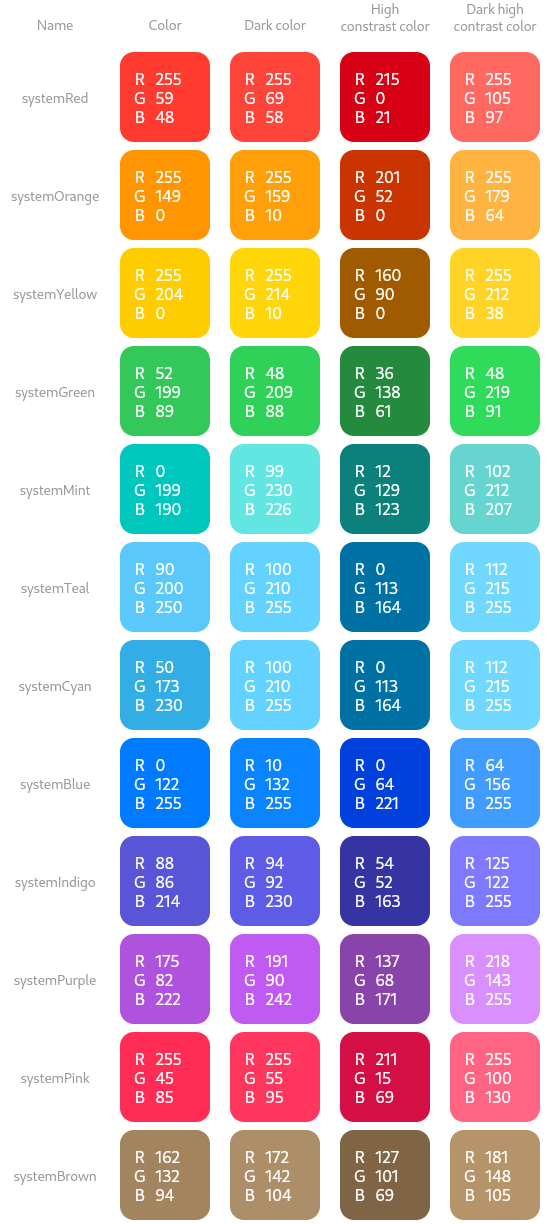
/// 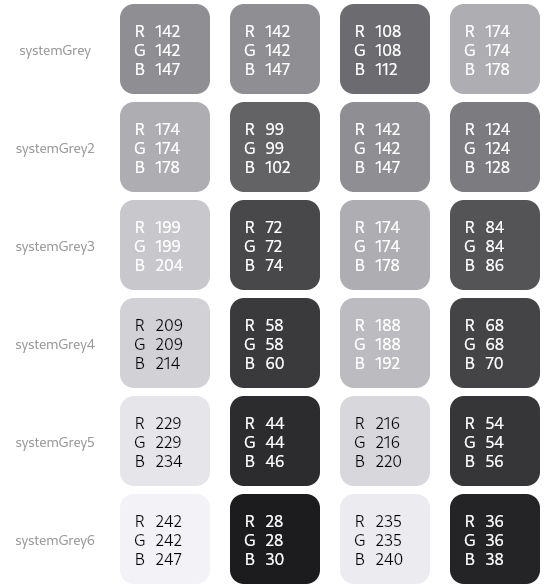
/// 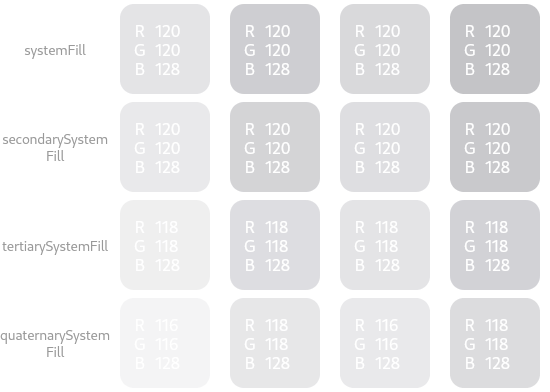
///
/// ### Label Colors
/// 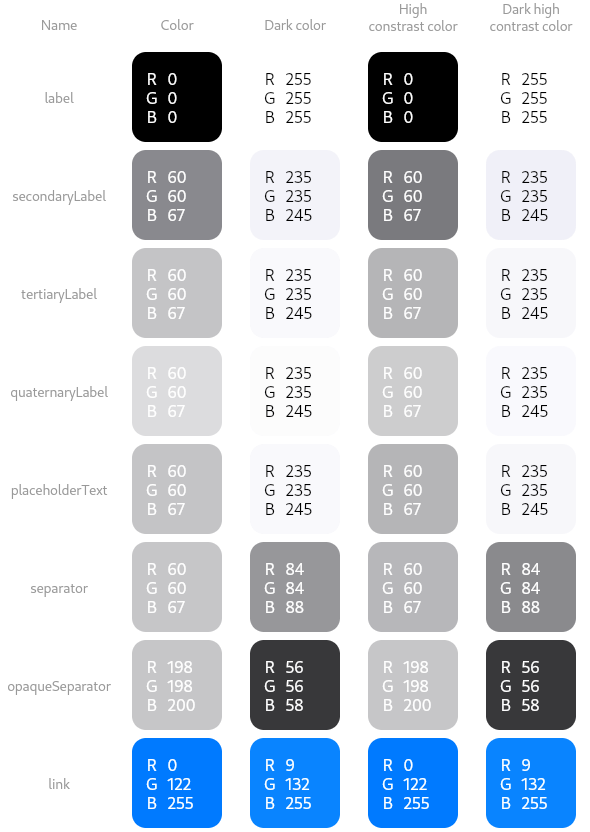
///
/// ### Background Colors
/// 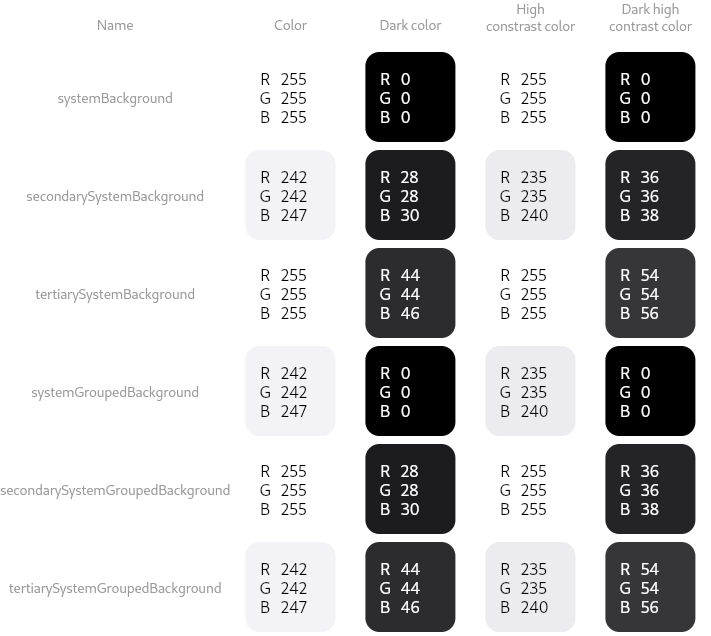
///
abstract final class CupertinoColors {
/// iOS 13's default blue color. Used to indicate active elements such as
/// buttons, selected tabs and your own chat bubbles.
///
/// This is SystemBlue in the iOS palette.
static const CupertinoDynamicColor activeBlue = systemBlue;
/// iOS 13's default green color. Used to indicate active accents such as
/// the switch in its on state and some accent buttons such as the call button
/// and Apple Map's 'Go' button.
///
/// This is SystemGreen in the iOS palette.
static const CupertinoDynamicColor activeGreen = systemGreen;
/// iOS 13's orange color.
///
/// This is SystemOrange in the iOS palette.
static const CupertinoDynamicColor activeOrange = systemOrange;
/// Opaque white color. Used for backgrounds and fonts against dark backgrounds.
///
/// This is SystemWhiteColor in the iOS palette.
///
/// See also:
///
/// * [material.Colors.white], the same color, in the Material Design palette.
/// * [black], opaque black in the [CupertinoColors] palette.
static const Color white = Color(0xFFFFFFFF);
/// Opaque black color. Used for texts against light backgrounds.
///
/// This is SystemBlackColor in the iOS palette.
///
/// See also:
///
/// * [material.Colors.black], the same color, in the Material Design palette.
/// * [white], opaque white in the [CupertinoColors] palette.
static const Color black = Color(0xFF000000);
/// Used in iOS 10 for light background fills such as the chat bubble background.
///
/// This is SystemLightGrayColor in the iOS palette.
static const Color lightBackgroundGray = Color(0xFFE5E5EA);
/// Used in iOS 12 for very light background fills in tables between cell groups.
///
/// This is SystemExtraLightGrayColor in the iOS palette.
static const Color extraLightBackgroundGray = Color(0xFFEFEFF4);
/// Used in iOS 12 for very dark background fills in tables between cell groups
/// in dark mode.
// Value derived from screenshot from the dark themed Apple Watch app.
static const Color darkBackgroundGray = Color(0xFF171717);
/// Used in iOS 13 for unselected selectables such as tab bar items in their
/// inactive state or de-emphasized subtitles and details text.
///
/// Not the same grey as disabled buttons etc.
///
/// This is the disabled color in the iOS palette.
static const CupertinoDynamicColor inactiveGray = CupertinoDynamicColor.withBrightness(
debugLabel: 'inactiveGray',
color: Color(0xFF999999),
darkColor: Color(0xFF757575),
);
/// Used for iOS 13 for destructive actions such as the delete actions in
/// table view cells and dialogs.
///
/// Not the same red as the camera shutter or springboard icon notifications
/// or the foreground red theme in various native apps such as HealthKit.
///
/// This is SystemRed in the iOS palette.
static const CupertinoDynamicColor destructiveRed = systemRed;
/// A blue color that can adapt to the given [BuildContext].
///
/// See also:
///
/// * [UIColor.systemBlue](https://developer.apple.com/documentation/uikit/uicolor/3173141-systemblue),
/// the `UIKit` equivalent.
static const CupertinoDynamicColor systemBlue = CupertinoDynamicColor.withBrightnessAndContrast(
debugLabel: 'systemBlue',
color: Color.fromARGB(255, 0, 122, 255),
darkColor: Color.fromARGB(255, 10, 132, 255),
highContrastColor: Color.fromARGB(255, 0, 64, 221),
darkHighContrastColor: Color.fromARGB(255, 64, 156, 255),
);
/// A green color that can adapt to the given [BuildContext].
///
/// See also:
///
/// * [UIColor.systemGreen](https://developer.apple.com/documentation/uikit/uicolor/3173144-systemgreen),
/// the `UIKit` equivalent.
static const CupertinoDynamicColor systemGreen = CupertinoDynamicColor.withBrightnessAndContrast(
debugLabel: 'systemGreen',
color: Color.fromARGB(255, 52, 199, 89),
darkColor: Color.fromARGB(255, 48, 209, 88),
highContrastColor: Color.fromARGB(255, 36, 138, 61),
darkHighContrastColor: Color.fromARGB(255, 48, 219, 91),
);
/// A mint color that can adapt to the given [BuildContext].
///
/// See also:
///
/// * [UIColor.systemMint](https://developer.apple.com/documentation/uikit/uicolor/3852741-systemmint),
/// the `UIKit` equivalent.
static const CupertinoDynamicColor systemMint =
CupertinoDynamicColor.withBrightnessAndContrast(
debugLabel: 'systemMint',
color: Color.fromARGB(255, 0, 199, 190),
darkColor: Color.fromARGB(255, 99, 230, 226),
highContrastColor: Color.fromARGB(255, 12, 129, 123),
darkHighContrastColor: Color.fromARGB(255, 102, 212, 207),
);
/// An indigo color that can adapt to the given [BuildContext].
///
/// See also:
///
/// * [UIColor.systemIndigo](https://developer.apple.com/documentation/uikit/uicolor/3173146-systemindigo),
/// the `UIKit` equivalent.
static const CupertinoDynamicColor systemIndigo = CupertinoDynamicColor.withBrightnessAndContrast(
debugLabel: 'systemIndigo',
color: Color.fromARGB(255, 88, 86, 214),
darkColor: Color.fromARGB(255, 94, 92, 230),
highContrastColor: Color.fromARGB(255, 54, 52, 163),
darkHighContrastColor: Color.fromARGB(255, 125, 122, 255),
);
/// An orange color that can adapt to the given [BuildContext].
///
/// See also:
///
/// * [UIColor.systemOrange](https://developer.apple.com/documentation/uikit/uicolor/3173147-systemorange),
/// the `UIKit` equivalent.
static const CupertinoDynamicColor systemOrange = CupertinoDynamicColor.withBrightnessAndContrast(
debugLabel: 'systemOrange',
color: Color.fromARGB(255, 255, 149, 0),
darkColor: Color.fromARGB(255, 255, 159, 10),
highContrastColor: Color.fromARGB(255, 201, 52, 0),
darkHighContrastColor: Color.fromARGB(255, 255, 179, 64),
);
/// A pink color that can adapt to the given [BuildContext].
///
/// See also:
///
/// * [UIColor.systemPink](https://developer.apple.com/documentation/uikit/uicolor/3173148-systempink),
/// the `UIKit` equivalent.
static const CupertinoDynamicColor systemPink = CupertinoDynamicColor.withBrightnessAndContrast(
debugLabel: 'systemPink',
color: Color.fromARGB(255, 255, 45, 85),
darkColor: Color.fromARGB(255, 255, 55, 95),
highContrastColor: Color.fromARGB(255, 211, 15, 69),
darkHighContrastColor: Color.fromARGB(255, 255, 100, 130),
);
/// A brown color that can adapt to the given [BuildContext].
///
/// See also:
///
/// * [UIColor.systemBrown](https://developer.apple.com/documentation/uikit/uicolor/3173142-systembrown),
/// the `UIKit` equivalent.
static const CupertinoDynamicColor systemBrown =
CupertinoDynamicColor.withBrightnessAndContrast(
debugLabel: 'systemBrown',
color: Color.fromARGB(255, 162, 132, 94),
darkColor: Color.fromARGB(255, 172, 142, 104),
highContrastColor: Color.fromARGB(255, 127, 101, 69),
darkHighContrastColor: Color.fromARGB(255, 181, 148, 105),
);
/// A purple color that can adapt to the given [BuildContext].
///
/// See also:
///
/// * [UIColor.systemPurple](https://developer.apple.com/documentation/uikit/uicolor/3173149-systempurple),
/// the `UIKit` equivalent.
static const CupertinoDynamicColor systemPurple = CupertinoDynamicColor.withBrightnessAndContrast(
debugLabel: 'systemPurple',
color: Color.fromARGB(255, 175, 82, 222),
darkColor: Color.fromARGB(255, 191, 90, 242),
highContrastColor: Color.fromARGB(255, 137, 68, 171),
darkHighContrastColor: Color.fromARGB(255, 218, 143, 255),
);
/// A red color that can adapt to the given [BuildContext].
///
/// See also:
///
/// * [UIColor.systemRed](https://developer.apple.com/documentation/uikit/uicolor/3173150-systemred),
/// the `UIKit` equivalent.
static const CupertinoDynamicColor systemRed = CupertinoDynamicColor.withBrightnessAndContrast(
debugLabel: 'systemRed',
color: Color.fromARGB(255, 255, 59, 48),
darkColor: Color.fromARGB(255, 255, 69, 58),
highContrastColor: Color.fromARGB(255, 215, 0, 21),
darkHighContrastColor: Color.fromARGB(255, 255, 105, 97),
);
/// A teal color that can adapt to the given [BuildContext].
///
/// See also:
///
/// * [UIColor.systemTeal](https://developer.apple.com/documentation/uikit/uicolor/3173151-systemteal),
/// the `UIKit` equivalent.
static const CupertinoDynamicColor systemTeal = CupertinoDynamicColor.withBrightnessAndContrast(
debugLabel: 'systemTeal',
color: Color.fromARGB(255, 90, 200, 250),
darkColor: Color.fromARGB(255, 100, 210, 255),
highContrastColor: Color.fromARGB(255, 0, 113, 164),
darkHighContrastColor: Color.fromARGB(255, 112, 215, 255),
);
/// A cyan color that can adapt to the given [BuildContext].
///
/// See also:
///
/// * [UIColor.systemCyan](https://developer.apple.com/documentation/uikit/uicolor/3852740-systemcyan),
/// the `UIKit` equivalent.
static const CupertinoDynamicColor systemCyan =
CupertinoDynamicColor.withBrightnessAndContrast(
debugLabel: 'systemCyan',
color: Color.fromARGB(255, 50, 173, 230),
darkColor: Color.fromARGB(255, 100, 210, 255),
highContrastColor: Color.fromARGB(255, 0, 113, 164),
darkHighContrastColor: Color.fromARGB(255, 112, 215, 255),
);
/// A yellow color that can adapt to the given [BuildContext].
///
/// See also:
///
/// * [UIColor.systemYellow](https://developer.apple.com/documentation/uikit/uicolor/3173152-systemyellow),
/// the `UIKit` equivalent.
static const CupertinoDynamicColor systemYellow = CupertinoDynamicColor.withBrightnessAndContrast(
debugLabel: 'systemYellow',
color: Color.fromARGB(255, 255, 204, 0),
darkColor: Color.fromARGB(255, 255, 214, 10),
highContrastColor: Color.fromARGB(255, 160, 90, 0),
darkHighContrastColor: Color.fromARGB(255, 255, 212, 38),
);
/// The base grey color.
///
/// See also:
///
/// * [UIColor.systemGray](https://developer.apple.com/documentation/uikit/uicolor/3173143-systemgray),
/// the `UIKit` equivalent.
static const CupertinoDynamicColor systemGrey = CupertinoDynamicColor.withBrightnessAndContrast(
debugLabel: 'systemGrey',
color: Color.fromARGB(255, 142, 142, 147),
darkColor: Color.fromARGB(255, 142, 142, 147),
highContrastColor: Color.fromARGB(255, 108, 108, 112),
darkHighContrastColor: Color.fromARGB(255, 174, 174, 178),
);
/// A second-level shade of grey.
///
/// See also:
///
/// * [UIColor.systemGray2](https://developer.apple.com/documentation/uikit/uicolor/3255071-systemgray2),
/// the `UIKit` equivalent.
static const CupertinoDynamicColor systemGrey2 = CupertinoDynamicColor.withBrightnessAndContrast(
debugLabel: 'systemGrey2',
color: Color.fromARGB(255, 174, 174, 178),
darkColor: Color.fromARGB(255, 99, 99, 102),
highContrastColor: Color.fromARGB(255, 142, 142, 147),
darkHighContrastColor: Color.fromARGB(255, 124, 124, 128),
);
/// A third-level shade of grey.
///
/// See also:
///
/// * [UIColor.systemGray3](https://developer.apple.com/documentation/uikit/uicolor/3255072-systemgray3),
/// the `UIKit` equivalent.
static const CupertinoDynamicColor systemGrey3 = CupertinoDynamicColor.withBrightnessAndContrast(
debugLabel: 'systemGrey3',
color: Color.fromARGB(255, 199, 199, 204),
darkColor: Color.fromARGB(255, 72, 72, 74),
highContrastColor: Color.fromARGB(255, 174, 174, 178),
darkHighContrastColor: Color.fromARGB(255, 84, 84, 86),
);
/// A fourth-level shade of grey.
///
/// See also:
///
/// * [UIColor.systemGray4](https://developer.apple.com/documentation/uikit/uicolor/3255073-systemgray4),
/// the `UIKit` equivalent.
static const CupertinoDynamicColor systemGrey4 = CupertinoDynamicColor.withBrightnessAndContrast(
debugLabel: 'systemGrey4',
color: Color.fromARGB(255, 209, 209, 214),
darkColor: Color.fromARGB(255, 58, 58, 60),
highContrastColor: Color.fromARGB(255, 188, 188, 192),
darkHighContrastColor: Color.fromARGB(255, 68, 68, 70),
);
/// A fifth-level shade of grey.
///
/// See also:
///
/// * [UIColor.systemGray5](https://developer.apple.com/documentation/uikit/uicolor/3255074-systemgray5),
/// the `UIKit` equivalent.
static const CupertinoDynamicColor systemGrey5 = CupertinoDynamicColor.withBrightnessAndContrast(
debugLabel: 'systemGrey5',
color: Color.fromARGB(255, 229, 229, 234),
darkColor: Color.fromARGB(255, 44, 44, 46),
highContrastColor: Color.fromARGB(255, 216, 216, 220),
darkHighContrastColor: Color.fromARGB(255, 54, 54, 56),
);
/// A sixth-level shade of grey.
///
/// See also:
///
/// * [UIColor.systemGray6](https://developer.apple.com/documentation/uikit/uicolor/3255075-systemgray6),
/// the `UIKit` equivalent.
static const CupertinoDynamicColor systemGrey6 = CupertinoDynamicColor.withBrightnessAndContrast(
debugLabel: 'systemGrey6',
color: Color.fromARGB(255, 242, 242, 247),
darkColor: Color.fromARGB(255, 28, 28, 30),
highContrastColor: Color.fromARGB(255, 235, 235, 240),
darkHighContrastColor: Color.fromARGB(255, 36, 36, 38),
);
/// The color for text labels containing primary content, equivalent to
/// [UIColor.label](https://developer.apple.com/documentation/uikit/uicolor/3173131-label).
static const CupertinoDynamicColor label = CupertinoDynamicColor(
debugLabel: 'label',
color: Color.fromARGB(255, 0, 0, 0),
darkColor: Color.fromARGB(255, 255, 255, 255),
highContrastColor: Color.fromARGB(255, 0, 0, 0),
darkHighContrastColor: Color.fromARGB(255, 255, 255, 255),
elevatedColor: Color.fromARGB(255, 0, 0, 0),
darkElevatedColor: Color.fromARGB(255, 255, 255, 255),
highContrastElevatedColor: Color.fromARGB(255, 0, 0, 0),
darkHighContrastElevatedColor: Color.fromARGB(255, 255, 255, 255),
);
/// The color for text labels containing secondary content, equivalent to
/// [UIColor.secondaryLabel](https://developer.apple.com/documentation/uikit/uicolor/3173136-secondarylabel).
static const CupertinoDynamicColor secondaryLabel = CupertinoDynamicColor(
debugLabel: 'secondaryLabel',
color: Color.fromARGB(153, 60, 60, 67),
darkColor: Color.fromARGB(153, 235, 235, 245),
highContrastColor: Color.fromARGB(173, 60, 60, 67),
darkHighContrastColor: Color.fromARGB(173, 235, 235, 245),
elevatedColor: Color.fromARGB(153, 60, 60, 67),
darkElevatedColor: Color.fromARGB(153, 235, 235, 245),
highContrastElevatedColor: Color.fromARGB(173, 60, 60, 67),
darkHighContrastElevatedColor: Color.fromARGB(173, 235, 235, 245),
);
/// The color for text labels containing tertiary content, equivalent to
/// [UIColor.tertiaryLabel](https://developer.apple.com/documentation/uikit/uicolor/3173153-tertiarylabel).
static const CupertinoDynamicColor tertiaryLabel = CupertinoDynamicColor(
debugLabel: 'tertiaryLabel',
color: Color.fromARGB(76, 60, 60, 67),
darkColor: Color.fromARGB(76, 235, 235, 245),
highContrastColor: Color.fromARGB(96, 60, 60, 67),
darkHighContrastColor: Color.fromARGB(96, 235, 235, 245),
elevatedColor: Color.fromARGB(76, 60, 60, 67),
darkElevatedColor: Color.fromARGB(76, 235, 235, 245),
highContrastElevatedColor: Color.fromARGB(96, 60, 60, 67),
darkHighContrastElevatedColor: Color.fromARGB(96, 235, 235, 245),
);
/// The color for text labels containing quaternary content, equivalent to
/// [UIColor.quaternaryLabel](https://developer.apple.com/documentation/uikit/uicolor/3173135-quaternarylabel).
static const CupertinoDynamicColor quaternaryLabel = CupertinoDynamicColor(
debugLabel: 'quaternaryLabel',
color: Color.fromARGB(45, 60, 60, 67),
darkColor: Color.fromARGB(40, 235, 235, 245),
highContrastColor: Color.fromARGB(66, 60, 60, 67),
darkHighContrastColor: Color.fromARGB(61, 235, 235, 245),
elevatedColor: Color.fromARGB(45, 60, 60, 67),
darkElevatedColor: Color.fromARGB(40, 235, 235, 245),
highContrastElevatedColor: Color.fromARGB(66, 60, 60, 67),
darkHighContrastElevatedColor: Color.fromARGB(61, 235, 235, 245),
);
/// An overlay fill color for thin and small shapes, equivalent to
/// [UIColor.systemFill](https://developer.apple.com/documentation/uikit/uicolor/3255070-systemfill).
static const CupertinoDynamicColor systemFill = CupertinoDynamicColor(
debugLabel: 'systemFill',
color: Color.fromARGB(51, 120, 120, 128),
darkColor: Color.fromARGB(91, 120, 120, 128),
highContrastColor: Color.fromARGB(71, 120, 120, 128),
darkHighContrastColor: Color.fromARGB(112, 120, 120, 128),
elevatedColor: Color.fromARGB(51, 120, 120, 128),
darkElevatedColor: Color.fromARGB(91, 120, 120, 128),
highContrastElevatedColor: Color.fromARGB(71, 120, 120, 128),
darkHighContrastElevatedColor: Color.fromARGB(112, 120, 120, 128),
);
/// An overlay fill color for medium-size shapes, equivalent to
/// [UIColor.secondarySystemFill](https://developer.apple.com/documentation/uikit/uicolor/3255069-secondarysystemfill).
static const CupertinoDynamicColor secondarySystemFill = CupertinoDynamicColor(
debugLabel: 'secondarySystemFill',
color: Color.fromARGB(40, 120, 120, 128),
darkColor: Color.fromARGB(81, 120, 120, 128),
highContrastColor: Color.fromARGB(61, 120, 120, 128),
darkHighContrastColor: Color.fromARGB(102, 120, 120, 128),
elevatedColor: Color.fromARGB(40, 120, 120, 128),
darkElevatedColor: Color.fromARGB(81, 120, 120, 128),
highContrastElevatedColor: Color.fromARGB(61, 120, 120, 128),
darkHighContrastElevatedColor: Color.fromARGB(102, 120, 120, 128),
);
/// An overlay fill color for large shapes, equivalent to
/// [UIColor.tertiarySystemFill](https://developer.apple.com/documentation/uikit/uicolor/3255076-tertiarysystemfill).
static const CupertinoDynamicColor tertiarySystemFill = CupertinoDynamicColor(
debugLabel: 'tertiarySystemFill',
color: Color.fromARGB(30, 118, 118, 128),
darkColor: Color.fromARGB(61, 118, 118, 128),
highContrastColor: Color.fromARGB(51, 118, 118, 128),
darkHighContrastColor: Color.fromARGB(81, 118, 118, 128),
elevatedColor: Color.fromARGB(30, 118, 118, 128),
darkElevatedColor: Color.fromARGB(61, 118, 118, 128),
highContrastElevatedColor: Color.fromARGB(51, 118, 118, 128),
darkHighContrastElevatedColor: Color.fromARGB(81, 118, 118, 128),
);
/// An overlay fill color for large areas containing complex content, equivalent
/// to [UIColor.quaternarySystemFill](https://developer.apple.com/documentation/uikit/uicolor/3255068-quaternarysystemfill).
static const CupertinoDynamicColor quaternarySystemFill = CupertinoDynamicColor(
debugLabel: 'quaternarySystemFill',
color: Color.fromARGB(20, 116, 116, 128),
darkColor: Color.fromARGB(45, 118, 118, 128),
highContrastColor: Color.fromARGB(40, 116, 116, 128),
darkHighContrastColor: Color.fromARGB(66, 118, 118, 128),
elevatedColor: Color.fromARGB(20, 116, 116, 128),
darkElevatedColor: Color.fromARGB(45, 118, 118, 128),
highContrastElevatedColor: Color.fromARGB(40, 116, 116, 128),
darkHighContrastElevatedColor: Color.fromARGB(66, 118, 118, 128),
);
/// The color for placeholder text in controls or text views, equivalent to
/// [UIColor.placeholderText](https://developer.apple.com/documentation/uikit/uicolor/3173134-placeholdertext).
static const CupertinoDynamicColor placeholderText = CupertinoDynamicColor(
debugLabel: 'placeholderText',
color: Color.fromARGB(76, 60, 60, 67),
darkColor: Color.fromARGB(76, 235, 235, 245),
highContrastColor: Color.fromARGB(96, 60, 60, 67),
darkHighContrastColor: Color.fromARGB(96, 235, 235, 245),
elevatedColor: Color.fromARGB(76, 60, 60, 67),
darkElevatedColor: Color.fromARGB(76, 235, 235, 245),
highContrastElevatedColor: Color.fromARGB(96, 60, 60, 67),
darkHighContrastElevatedColor: Color.fromARGB(96, 235, 235, 245),
);
/// The color for the main background of your interface, equivalent to
/// [UIColor.systemBackground](https://developer.apple.com/documentation/uikit/uicolor/3173140-systembackground).
///
/// Typically used for designs that have a white primary background in a light environment.
static const CupertinoDynamicColor systemBackground = CupertinoDynamicColor(
debugLabel: 'systemBackground',
color: Color.fromARGB(255, 255, 255, 255),
darkColor: Color.fromARGB(255, 0, 0, 0),
highContrastColor: Color.fromARGB(255, 255, 255, 255),
darkHighContrastColor: Color.fromARGB(255, 0, 0, 0),
elevatedColor: Color.fromARGB(255, 255, 255, 255),
darkElevatedColor: Color.fromARGB(255, 28, 28, 30),
highContrastElevatedColor: Color.fromARGB(255, 255, 255, 255),
darkHighContrastElevatedColor: Color.fromARGB(255, 36, 36, 38),
);
/// The color for content layered on top of the main background, equivalent to
/// [UIColor.secondarySystemBackground](https://developer.apple.com/documentation/uikit/uicolor/3173137-secondarysystembackground).
///
/// Typically used for designs that have a white primary background in a light environment.
static const CupertinoDynamicColor secondarySystemBackground = CupertinoDynamicColor(
debugLabel: 'secondarySystemBackground',
color: Color.fromARGB(255, 242, 242, 247),
darkColor: Color.fromARGB(255, 28, 28, 30),
highContrastColor: Color.fromARGB(255, 235, 235, 240),
darkHighContrastColor: Color.fromARGB(255, 36, 36, 38),
elevatedColor: Color.fromARGB(255, 242, 242, 247),
darkElevatedColor: Color.fromARGB(255, 44, 44, 46),
highContrastElevatedColor: Color.fromARGB(255, 235, 235, 240),
darkHighContrastElevatedColor: Color.fromARGB(255, 54, 54, 56),
);
/// The color for content layered on top of secondary backgrounds, equivalent
/// to [UIColor.tertiarySystemBackground](https://developer.apple.com/documentation/uikit/uicolor/3173154-tertiarysystembackground).
///
/// Typically used for designs that have a white primary background in a light environment.
static const CupertinoDynamicColor tertiarySystemBackground = CupertinoDynamicColor(
debugLabel: 'tertiarySystemBackground',
color: Color.fromARGB(255, 255, 255, 255),
darkColor: Color.fromARGB(255, 44, 44, 46),
highContrastColor: Color.fromARGB(255, 255, 255, 255),
darkHighContrastColor: Color.fromARGB(255, 54, 54, 56),
elevatedColor: Color.fromARGB(255, 255, 255, 255),
darkElevatedColor: Color.fromARGB(255, 58, 58, 60),
highContrastElevatedColor: Color.fromARGB(255, 255, 255, 255),
darkHighContrastElevatedColor: Color.fromARGB(255, 68, 68, 70),
);
/// The color for the main background of your grouped interface, equivalent to
/// [UIColor.systemGroupedBackground](https://developer.apple.com/documentation/uikit/uicolor/3173145-systemgroupedbackground).
///
/// Typically used for grouped content, including table views and platter-based designs.
static const CupertinoDynamicColor systemGroupedBackground = CupertinoDynamicColor(
debugLabel: 'systemGroupedBackground',
color: Color.fromARGB(255, 242, 242, 247),
darkColor: Color.fromARGB(255, 0, 0, 0),
highContrastColor: Color.fromARGB(255, 235, 235, 240),
darkHighContrastColor: Color.fromARGB(255, 0, 0, 0),
elevatedColor: Color.fromARGB(255, 242, 242, 247),
darkElevatedColor: Color.fromARGB(255, 28, 28, 30),
highContrastElevatedColor: Color.fromARGB(255, 235, 235, 240),
darkHighContrastElevatedColor: Color.fromARGB(255, 36, 36, 38),
);
/// The color for content layered on top of the main background of your grouped interface,
/// equivalent to [UIColor.secondarySystemGroupedBackground](https://developer.apple.com/documentation/uikit/uicolor/3173138-secondarysystemgroupedbackground).
///
/// Typically used for grouped content, including table views and platter-based designs.
static const CupertinoDynamicColor secondarySystemGroupedBackground = CupertinoDynamicColor(
debugLabel: 'secondarySystemGroupedBackground',
color: Color.fromARGB(255, 255, 255, 255),
darkColor: Color.fromARGB(255, 28, 28, 30),
highContrastColor: Color.fromARGB(255, 255, 255, 255),
darkHighContrastColor: Color.fromARGB(255, 36, 36, 38),
elevatedColor: Color.fromARGB(255, 255, 255, 255),
darkElevatedColor: Color.fromARGB(255, 44, 44, 46),
highContrastElevatedColor: Color.fromARGB(255, 255, 255, 255),
darkHighContrastElevatedColor: Color.fromARGB(255, 54, 54, 56),
);
/// The color for content layered on top of secondary backgrounds of your grouped interface,
/// equivalent to [UIColor.tertiarySystemGroupedBackground](https://developer.apple.com/documentation/uikit/uicolor/3173155-tertiarysystemgroupedbackground).
///
/// Typically used for grouped content, including table views and platter-based designs.
static const CupertinoDynamicColor tertiarySystemGroupedBackground = CupertinoDynamicColor(
debugLabel: 'tertiarySystemGroupedBackground',
color: Color.fromARGB(255, 242, 242, 247),
darkColor: Color.fromARGB(255, 44, 44, 46),
highContrastColor: Color.fromARGB(255, 235, 235, 240),
darkHighContrastColor: Color.fromARGB(255, 54, 54, 56),
elevatedColor: Color.fromARGB(255, 242, 242, 247),
darkElevatedColor: Color.fromARGB(255, 58, 58, 60),
highContrastElevatedColor: Color.fromARGB(255, 235, 235, 240),
darkHighContrastElevatedColor: Color.fromARGB(255, 68, 68, 70),
);
/// The color for thin borders or divider lines that allows some underlying content to be visible,
/// equivalent to [UIColor.separator](https://developer.apple.com/documentation/uikit/uicolor/3173139-separator).
static const CupertinoDynamicColor separator = CupertinoDynamicColor(
debugLabel: 'separator',
color: Color.fromARGB(73, 60, 60, 67),
darkColor: Color.fromARGB(153, 84, 84, 88),
highContrastColor: Color.fromARGB(94, 60, 60, 67),
darkHighContrastColor: Color.fromARGB(173, 84, 84, 88),
elevatedColor: Color.fromARGB(73, 60, 60, 67),
darkElevatedColor: Color.fromARGB(153, 84, 84, 88),
highContrastElevatedColor: Color.fromARGB(94, 60, 60, 67),
darkHighContrastElevatedColor: Color.fromARGB(173, 84, 84, 88),
);
/// The color for borders or divider lines that hide any underlying content,
/// equivalent to [UIColor.opaqueSeparator](https://developer.apple.com/documentation/uikit/uicolor/3173133-opaqueseparator).
static const CupertinoDynamicColor opaqueSeparator = CupertinoDynamicColor(
debugLabel: 'opaqueSeparator',
color: Color.fromARGB(255, 198, 198, 200),
darkColor: Color.fromARGB(255, 56, 56, 58),
highContrastColor: Color.fromARGB(255, 198, 198, 200),
darkHighContrastColor: Color.fromARGB(255, 56, 56, 58),
elevatedColor: Color.fromARGB(255, 198, 198, 200),
darkElevatedColor: Color.fromARGB(255, 56, 56, 58),
highContrastElevatedColor: Color.fromARGB(255, 198, 198, 200),
darkHighContrastElevatedColor: Color.fromARGB(255, 56, 56, 58),
);
/// The color for links, equivalent to
/// [UIColor.link](https://developer.apple.com/documentation/uikit/uicolor/3173132-link).
static const CupertinoDynamicColor link = CupertinoDynamicColor(
debugLabel: 'link',
color: Color.fromARGB(255, 0, 122, 255),
darkColor: Color.fromARGB(255, 9, 132, 255),
highContrastColor: Color.fromARGB(255, 0, 122, 255),
darkHighContrastColor: Color.fromARGB(255, 9, 132, 255),
elevatedColor: Color.fromARGB(255, 0, 122, 255),
darkElevatedColor: Color.fromARGB(255, 9, 132, 255),
highContrastElevatedColor: Color.fromARGB(255, 0, 122, 255),
darkHighContrastElevatedColor: Color.fromARGB(255, 9, 132, 255),
);
}
/// A [Color] subclass that represents a family of colors, and the correct effective
/// color in the color family.
///
/// When used as a regular color, [CupertinoDynamicColor] is equivalent to the
/// effective color (i.e. [CupertinoDynamicColor.value] will come from the effective
/// color), which is determined by the [BuildContext] it is last resolved against.
/// If it has never been resolved, the light, normal contrast, base elevation variant
/// [CupertinoDynamicColor.color] will be the default effective color.
///
/// Sometimes manually resolving a [CupertinoDynamicColor] is not necessary, because
/// the Cupertino Library provides built-in support for it.
///
/// ### Using [CupertinoDynamicColor] in a Cupertino widget
///
/// When a Cupertino widget is provided with a [CupertinoDynamicColor], either
/// directly in its constructor, or from an [InheritedWidget] it depends on (for example,
/// [DefaultTextStyle]), the widget will automatically resolve the color using
/// [CupertinoDynamicColor.resolve] against its own [BuildContext], on a best-effort
/// basis.
///
/// {@tool snippet}
/// By default a [CupertinoButton] has no background color. The following sample
/// code shows how to build a [CupertinoButton] that appears white in light mode,
/// and changes automatically to black in dark mode.
///
/// ```dart
/// CupertinoButton(
/// // CupertinoDynamicColor works out of box in a CupertinoButton.
/// color: const CupertinoDynamicColor.withBrightness(
/// color: CupertinoColors.white,
/// darkColor: CupertinoColors.black,
/// ),
/// onPressed: () { },
/// child: child,
/// )
/// ```
/// {@end-tool}
///
/// ### Using a [CupertinoDynamicColor] from a [CupertinoTheme]
///
/// When referring to a [CupertinoTheme] color, generally the color will already
/// have adapted to the ambient [BuildContext], because [CupertinoTheme.of]
/// implicitly resolves all the colors used in the retrieved [CupertinoThemeData],
/// before returning it.
///
/// {@tool snippet}
/// The following code sample creates a [Container] with the `primaryColor` of the
/// current theme. If `primaryColor` is a [CupertinoDynamicColor], the container
/// will be adaptive, thanks to [CupertinoTheme.of]: it will switch to `primaryColor`'s
/// dark variant once dark mode is turned on, and turns to primaryColor`'s high
/// contrast variant when [MediaQueryData.highContrast] is requested in the ambient
/// [MediaQuery], etc.
///
/// ```dart
/// Container(
/// // Container is not a Cupertino widget, but CupertinoTheme.of implicitly
/// // resolves colors used in the retrieved CupertinoThemeData.
/// color: CupertinoTheme.of(context).primaryColor,
/// )
/// ```
/// {@end-tool}
///
/// ### Manually Resolving a [CupertinoDynamicColor]
///
/// When used to configure a non-Cupertino widget, or wrapped in an object opaque
/// to the receiving Cupertino component, a [CupertinoDynamicColor] may need to be
/// manually resolved using [CupertinoDynamicColor.resolve], before it can used
/// to paint. For example, to use a custom [Border] in a [CupertinoNavigationBar],
/// the colors used in the [Border] have to be resolved manually before being passed
/// to [CupertinoNavigationBar]'s constructor.
///
/// {@tool snippet}
///
/// The following code samples demonstrate two cases where you have to manually
/// resolve a [CupertinoDynamicColor].
///
/// ```dart
/// CupertinoNavigationBar(
/// // CupertinoNavigationBar does not know how to resolve colors used in
/// // a Border class.
/// border: Border(
/// bottom: BorderSide(
/// color: CupertinoDynamicColor.resolve(CupertinoColors.systemBlue, context),
/// ),
/// ),
/// )
/// ```
///
/// ```dart
/// Container(
/// // Container is not a Cupertino widget.
/// color: CupertinoDynamicColor.resolve(CupertinoColors.systemBlue, context),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [CupertinoUserInterfaceLevel], an [InheritedWidget] that may affect color
/// resolution of a [CupertinoDynamicColor].
/// * [CupertinoTheme.of], a static method that retrieves the ambient [CupertinoThemeData],
/// and then resolves [CupertinoDynamicColor]s used in the retrieved data.
@immutable
class CupertinoDynamicColor extends Color with Diagnosticable {
/// Creates an adaptive [Color] that changes its effective color based on the
/// [BuildContext] given. The default effective color is [color].
const CupertinoDynamicColor({
String? debugLabel,
required Color color,
required Color darkColor,
required Color highContrastColor,
required Color darkHighContrastColor,
required Color elevatedColor,
required Color darkElevatedColor,
required Color highContrastElevatedColor,
required Color darkHighContrastElevatedColor,
}) : this._(
color,
color,
darkColor,
highContrastColor,
darkHighContrastColor,
elevatedColor,
darkElevatedColor,
highContrastElevatedColor,
darkHighContrastElevatedColor,
null,
debugLabel,
);
/// Creates an adaptive [Color] that changes its effective color based on the
/// given [BuildContext]'s brightness (from [MediaQueryData.platformBrightness]
/// or [CupertinoThemeData.brightness]) and accessibility contrast setting
/// ([MediaQueryData.highContrast]). The default effective color is [color].
const CupertinoDynamicColor.withBrightnessAndContrast({
String? debugLabel,
required Color color,
required Color darkColor,
required Color highContrastColor,
required Color darkHighContrastColor,
}) : this(
debugLabel: debugLabel,
color: color,
darkColor: darkColor,
highContrastColor: highContrastColor,
darkHighContrastColor: darkHighContrastColor,
elevatedColor: color,
darkElevatedColor: darkColor,
highContrastElevatedColor: highContrastColor,
darkHighContrastElevatedColor: darkHighContrastColor,
);
/// Creates an adaptive [Color] that changes its effective color based on the given
/// [BuildContext]'s brightness (from [MediaQueryData.platformBrightness] or
/// [CupertinoThemeData.brightness]). The default effective color is [color].
const CupertinoDynamicColor.withBrightness({
String? debugLabel,
required Color color,
required Color darkColor,
}) : this(
debugLabel: debugLabel,
color: color,
darkColor: darkColor,
highContrastColor: color,
darkHighContrastColor: darkColor,
elevatedColor: color,
darkElevatedColor: darkColor,
highContrastElevatedColor: color,
darkHighContrastElevatedColor: darkColor,
);
const CupertinoDynamicColor._(
this._effectiveColor,
this.color,
this.darkColor,
this.highContrastColor,
this.darkHighContrastColor,
this.elevatedColor,
this.darkElevatedColor,
this.highContrastElevatedColor,
this.darkHighContrastElevatedColor,
this._debugResolveContext,
this._debugLabel,
) : // The super constructor has to be called with a dummy value in order to mark
// this constructor const.
// The field `value` is overridden in the class implementation.
super(0);
/// The current effective color.
///
/// Defaults to [color] if this [CupertinoDynamicColor] has never been
/// resolved.
final Color _effectiveColor;
@override
int get value => _effectiveColor.value;
final String? _debugLabel;
final Element? _debugResolveContext;
/// The color to use when the [BuildContext] implies a combination of light mode,
/// normal contrast, and base interface elevation.
///
/// In other words, this color will be the effective color of the [CupertinoDynamicColor]
/// after it is resolved against a [BuildContext] that:
/// - has a [CupertinoTheme] whose [CupertinoThemeData.brightness] is [Brightness.light],
/// or a [MediaQuery] whose [MediaQueryData.platformBrightness] is [Brightness.light].
/// - has a [MediaQuery] whose [MediaQueryData.highContrast] is `false`.
/// - has a [CupertinoUserInterfaceLevel] that indicates [CupertinoUserInterfaceLevelData.base].
final Color color;
/// The color to use when the [BuildContext] implies a combination of dark mode,
/// normal contrast, and base interface elevation.
///
/// In other words, this color will be the effective color of the [CupertinoDynamicColor]
/// after it is resolved against a [BuildContext] that:
/// - has a [CupertinoTheme] whose [CupertinoThemeData.brightness] is [Brightness.dark],
/// or a [MediaQuery] whose [MediaQueryData.platformBrightness] is [Brightness.dark].
/// - has a [MediaQuery] whose [MediaQueryData.highContrast] is `false`.
/// - has a [CupertinoUserInterfaceLevel] that indicates [CupertinoUserInterfaceLevelData.base].
final Color darkColor;
/// The color to use when the [BuildContext] implies a combination of light mode,
/// high contrast, and base interface elevation.
///
/// In other words, this color will be the effective color of the [CupertinoDynamicColor]
/// after it is resolved against a [BuildContext] that:
/// - has a [CupertinoTheme] whose [CupertinoThemeData.brightness] is [Brightness.light],
/// or a [MediaQuery] whose [MediaQueryData.platformBrightness] is [Brightness.light].
/// - has a [MediaQuery] whose [MediaQueryData.highContrast] is `true`.
/// - has a [CupertinoUserInterfaceLevel] that indicates [CupertinoUserInterfaceLevelData.base].
final Color highContrastColor;
/// The color to use when the [BuildContext] implies a combination of dark mode,
/// high contrast, and base interface elevation.
///
/// In other words, this color will be the effective color of the [CupertinoDynamicColor]
/// after it is resolved against a [BuildContext] that:
/// - has a [CupertinoTheme] whose [CupertinoThemeData.brightness] is [Brightness.dark],
/// or a [MediaQuery] whose [MediaQueryData.platformBrightness] is [Brightness.dark].
/// - has a [MediaQuery] whose [MediaQueryData.highContrast] is `true`.
/// - has a [CupertinoUserInterfaceLevel] that indicates [CupertinoUserInterfaceLevelData.base].
final Color darkHighContrastColor;
/// The color to use when the [BuildContext] implies a combination of light mode,
/// normal contrast, and elevated interface elevation.
///
/// In other words, this color will be the effective color of the [CupertinoDynamicColor]
/// after it is resolved against a [BuildContext] that:
/// - has a [CupertinoTheme] whose [CupertinoThemeData.brightness] is [Brightness.light],
/// or a [MediaQuery] whose [MediaQueryData.platformBrightness] is [Brightness.light].
/// - has a [MediaQuery] whose [MediaQueryData.highContrast] is `false`.
/// - has a [CupertinoUserInterfaceLevel] that indicates [CupertinoUserInterfaceLevelData.elevated].
final Color elevatedColor;
/// The color to use when the [BuildContext] implies a combination of dark mode,
/// normal contrast, and elevated interface elevation.
///
/// In other words, this color will be the effective color of the [CupertinoDynamicColor]
/// after it is resolved against a [BuildContext] that:
/// - has a [CupertinoTheme] whose [CupertinoThemeData.brightness] is [Brightness.dark],
/// or a [MediaQuery] whose [MediaQueryData.platformBrightness] is [Brightness.dark].
/// - has a [MediaQuery] whose [MediaQueryData.highContrast] is `false`.
/// - has a [CupertinoUserInterfaceLevel] that indicates [CupertinoUserInterfaceLevelData.elevated].
final Color darkElevatedColor;
/// The color to use when the [BuildContext] implies a combination of light mode,
/// high contrast, and elevated interface elevation.
///
/// In other words, this color will be the effective color of the [CupertinoDynamicColor]
/// after it is resolved against a [BuildContext] that:
/// - has a [CupertinoTheme] whose [CupertinoThemeData.brightness] is [Brightness.light],
/// or a [MediaQuery] whose [MediaQueryData.platformBrightness] is [Brightness.light].
/// - has a [MediaQuery] whose [MediaQueryData.highContrast] is `true`.
/// - has a [CupertinoUserInterfaceLevel] that indicates [CupertinoUserInterfaceLevelData.elevated].
final Color highContrastElevatedColor;
/// The color to use when the [BuildContext] implies a combination of dark mode,
/// high contrast, and elevated interface elevation.
///
/// In other words, this color will be the effective color of the [CupertinoDynamicColor]
/// after it is resolved against a [BuildContext] that:
/// - has a [CupertinoTheme] whose [CupertinoThemeData.brightness] is [Brightness.dark],
/// or a [MediaQuery] whose [MediaQueryData.platformBrightness] is [Brightness.dark].
/// - has a [MediaQuery] whose [MediaQueryData.highContrast] is `true`.
/// - has a [CupertinoUserInterfaceLevel] that indicates [CupertinoUserInterfaceLevelData.elevated].
final Color darkHighContrastElevatedColor;
/// Resolves the given [Color] by calling [resolveFrom].
///
/// If the given color is already a concrete [Color], it will be returned as is.
/// If the given color is a [CupertinoDynamicColor], but the given [BuildContext]
/// lacks the dependencies required to the color resolution, the default trait
/// value will be used ([Brightness.light] platform brightness, normal contrast,
/// [CupertinoUserInterfaceLevelData.base] elevation level).
///
/// See also:
///
/// * [maybeResolve], which is similar to this function, but will allow a
/// null `resolvable` color.
static Color resolve(Color resolvable, BuildContext context) {
return (resolvable is CupertinoDynamicColor)
? resolvable.resolveFrom(context)
: resolvable;
}
/// Resolves the given [Color] by calling [resolveFrom].
///
/// If the given color is already a concrete [Color], it will be returned as is.
/// If the given color is null, returns null.
/// If the given color is a [CupertinoDynamicColor], but the given [BuildContext]
/// lacks the dependencies required to the color resolution, the default trait
/// value will be used ([Brightness.light] platform brightness, normal contrast,
/// [CupertinoUserInterfaceLevelData.base] elevation level).
///
/// See also:
///
/// * [resolve], which is similar to this function, but returns a
/// non-nullable value, and does not allow a null `resolvable` color.
static Color? maybeResolve(Color? resolvable, BuildContext context) {
return (resolvable is CupertinoDynamicColor)
? resolvable.resolveFrom(context)
: resolvable;
}
bool get _isPlatformBrightnessDependent {
return color != darkColor
|| elevatedColor != darkElevatedColor
|| highContrastColor != darkHighContrastColor
|| highContrastElevatedColor != darkHighContrastElevatedColor;
}
bool get _isHighContrastDependent {
return color != highContrastColor
|| darkColor != darkHighContrastColor
|| elevatedColor != highContrastElevatedColor
|| darkElevatedColor != darkHighContrastElevatedColor;
}
bool get _isInterfaceElevationDependent {
return color != elevatedColor
|| darkColor != darkElevatedColor
|| highContrastColor != highContrastElevatedColor
|| darkHighContrastColor != darkHighContrastElevatedColor;
}
/// Resolves this [CupertinoDynamicColor] using the provided [BuildContext].
///
/// Calling this method will create a new [CupertinoDynamicColor] that is
/// almost identical to this [CupertinoDynamicColor], except the effective
/// color is changed to adapt to the given [BuildContext].
///
/// For example, if the given [BuildContext] indicates the widgets in the
/// subtree should be displayed in dark mode (the surrounding
/// [CupertinoTheme]'s [CupertinoThemeData.brightness] or [MediaQuery]'s
/// [MediaQueryData.platformBrightness] is [Brightness.dark]), with a high
/// accessibility contrast (the surrounding [MediaQuery]'s
/// [MediaQueryData.highContrast] is `true`), and an elevated interface
/// elevation (the surrounding [CupertinoUserInterfaceLevel]'s `data` is
/// [CupertinoUserInterfaceLevelData.elevated]), the resolved
/// [CupertinoDynamicColor] will be the same as this [CupertinoDynamicColor],
/// except its effective color will be the `darkHighContrastElevatedColor`
/// variant from the original [CupertinoDynamicColor].
///
/// Calling this function may create dependencies on the closest instance of
/// some [InheritedWidget]s that enclose the given [BuildContext]. E.g., if
/// [darkColor] is different from [color], this method will call
/// [CupertinoTheme.maybeBrightnessOf] in an effort to determine the
/// brightness. If [color] is different from [highContrastColor], this method
/// will call [MediaQuery.maybeHighContrastOf] in an effort to determine the
/// high contrast setting.
///
/// If any of the required dependencies are missing from the given context,
/// the default value of that trait will be used ([Brightness.light] platform
/// brightness, normal contrast, [CupertinoUserInterfaceLevelData.base]
/// elevation level).
CupertinoDynamicColor resolveFrom(BuildContext context) {
final Brightness brightness = _isPlatformBrightnessDependent
? CupertinoTheme.maybeBrightnessOf(context) ?? Brightness.light
: Brightness.light;
final CupertinoUserInterfaceLevelData level = _isInterfaceElevationDependent
? CupertinoUserInterfaceLevel.maybeOf(context) ?? CupertinoUserInterfaceLevelData.base
: CupertinoUserInterfaceLevelData.base;
final bool highContrast = _isHighContrastDependent
&& (MediaQuery.maybeHighContrastOf(context) ?? false);
final Color resolved = switch ((brightness, level, highContrast)) {
(Brightness.light, CupertinoUserInterfaceLevelData.base, false) => color,
(Brightness.light, CupertinoUserInterfaceLevelData.base, true) => highContrastColor,
(Brightness.light, CupertinoUserInterfaceLevelData.elevated, false) => elevatedColor,
(Brightness.light, CupertinoUserInterfaceLevelData.elevated, true) => highContrastElevatedColor,
(Brightness.dark, CupertinoUserInterfaceLevelData.base, false) => darkColor,
(Brightness.dark, CupertinoUserInterfaceLevelData.base, true) => darkHighContrastColor,
(Brightness.dark, CupertinoUserInterfaceLevelData.elevated, false) => darkElevatedColor,
(Brightness.dark, CupertinoUserInterfaceLevelData.elevated, true) => darkHighContrastElevatedColor,
};
Element? debugContext;
assert(() {
debugContext = context as Element;
return true;
}());
return CupertinoDynamicColor._(
resolved,
color,
darkColor,
highContrastColor,
darkHighContrastColor,
elevatedColor,
darkElevatedColor,
highContrastElevatedColor,
darkHighContrastElevatedColor,
debugContext,
_debugLabel,
);
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is CupertinoDynamicColor
&& other.value == value
&& other.color == color
&& other.darkColor == darkColor
&& other.highContrastColor == highContrastColor
&& other.darkHighContrastColor == darkHighContrastColor
&& other.elevatedColor == elevatedColor
&& other.darkElevatedColor == darkElevatedColor
&& other.highContrastElevatedColor == highContrastElevatedColor
&& other.darkHighContrastElevatedColor == darkHighContrastElevatedColor;
}
@override
int get hashCode => Object.hash(
value,
color,
darkColor,
highContrastColor,
elevatedColor,
darkElevatedColor,
darkHighContrastColor,
darkHighContrastElevatedColor,
highContrastElevatedColor,
);
@override
String toString({ DiagnosticLevel minLevel = DiagnosticLevel.info }) {
String toString(String name, Color color) {
final String marker = color == _effectiveColor ? '*' : '';
return '$marker$name = $color$marker';
}
final List<String> xs = <String>[toString('color', color),
if (_isPlatformBrightnessDependent) toString('darkColor', darkColor),
if (_isHighContrastDependent) toString('highContrastColor', highContrastColor),
if (_isPlatformBrightnessDependent && _isHighContrastDependent) toString('darkHighContrastColor', darkHighContrastColor),
if (_isInterfaceElevationDependent) toString('elevatedColor', elevatedColor),
if (_isPlatformBrightnessDependent && _isInterfaceElevationDependent) toString('darkElevatedColor', darkElevatedColor),
if (_isHighContrastDependent && _isInterfaceElevationDependent) toString('highContrastElevatedColor', highContrastElevatedColor),
if (_isPlatformBrightnessDependent && _isHighContrastDependent && _isInterfaceElevationDependent) toString('darkHighContrastElevatedColor', darkHighContrastElevatedColor),
];
return '${_debugLabel ?? objectRuntimeType(this, 'CupertinoDynamicColor')}(${xs.join(', ')}, resolved by: ${_debugResolveContext?.widget ?? "UNRESOLVED"})';
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
if (_debugLabel != null) {
properties.add(MessageProperty('debugLabel', _debugLabel));
}
properties.add(createCupertinoColorProperty('color', color));
if (_isPlatformBrightnessDependent) {
properties.add(createCupertinoColorProperty('darkColor', darkColor));
}
if (_isHighContrastDependent) {
properties.add(createCupertinoColorProperty('highContrastColor', highContrastColor));
}
if (_isPlatformBrightnessDependent && _isHighContrastDependent) {
properties.add(createCupertinoColorProperty('darkHighContrastColor', darkHighContrastColor));
}
if (_isInterfaceElevationDependent) {
properties.add(createCupertinoColorProperty('elevatedColor', elevatedColor));
}
if (_isPlatformBrightnessDependent && _isInterfaceElevationDependent) {
properties.add(createCupertinoColorProperty('darkElevatedColor', darkElevatedColor));
}
if (_isHighContrastDependent && _isInterfaceElevationDependent) {
properties.add(createCupertinoColorProperty('highContrastElevatedColor', highContrastElevatedColor));
}
if (_isPlatformBrightnessDependent && _isHighContrastDependent && _isInterfaceElevationDependent) {
properties.add(createCupertinoColorProperty('darkHighContrastElevatedColor', darkHighContrastElevatedColor));
}
if (_debugResolveContext != null) {
properties.add(DiagnosticsProperty<Element>('last resolved', _debugResolveContext));
}
}
}
/// Creates a diagnostics property for [CupertinoDynamicColor].
DiagnosticsProperty<Color> createCupertinoColorProperty(
String name,
Color? value, {
bool showName = true,
Object? defaultValue = kNoDefaultValue,
DiagnosticsTreeStyle style = DiagnosticsTreeStyle.singleLine,
DiagnosticLevel level = DiagnosticLevel.info,
}) {
if (value is CupertinoDynamicColor) {
return DiagnosticsProperty<CupertinoDynamicColor>(
name,
value,
description: value._debugLabel,
showName: showName,
defaultValue: defaultValue,
style: style,
level: level,
);
} else {
return ColorProperty(
name,
value,
showName: showName,
defaultValue: defaultValue,
style: style,
level: level,
);
}
}
| flutter/packages/flutter/lib/src/cupertino/colors.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/colors.dart",
"repo_id": "flutter",
"token_count": 17431
} | 640 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/widgets.dart';
import 'colors.dart';
import 'icons.dart';
import 'theme.dart';
// These constants were eyeballed from iOS 14.4 Settings app for base, Notes for
// notched without leading, and Reminders app for notched with leading.
const double _kLeadingSize = 28.0;
const double _kNotchedLeadingSize = 30.0;
const double _kMinHeight = _kLeadingSize + 2 * 8.0;
const double _kMinHeightWithSubtitle = _kLeadingSize + 2 * 10.0;
const double _kNotchedMinHeight = _kNotchedLeadingSize + 2 * 12.0;
const double _kNotchedMinHeightWithoutLeading = _kNotchedLeadingSize + 2 * 10.0;
const EdgeInsetsDirectional _kPadding = EdgeInsetsDirectional.only(start: 20.0, end: 14.0);
const EdgeInsetsDirectional _kPaddingWithSubtitle = EdgeInsetsDirectional.only(start: 20.0, end: 14.0);
const EdgeInsets _kNotchedPadding = EdgeInsets.symmetric(horizontal: 14.0);
const EdgeInsetsDirectional _kNotchedPaddingWithoutLeading = EdgeInsetsDirectional.fromSTEB(28.0, 10.0, 14.0, 10.0);
const double _kLeadingToTitle = 16.0;
const double _kNotchedLeadingToTitle = 12.0;
const double _kNotchedTitleToSubtitle = 3.0;
const double _kAdditionalInfoToTrailing = 6.0;
const double _kNotchedTitleWithSubtitleFontSize = 16.0;
const double _kSubtitleFontSize = 12.0;
const double _kNotchedSubtitleFontSize = 14.0;
enum _CupertinoListTileType { base, notched }
/// An iOS-style list tile.
///
/// The [CupertinoListTile] is a Cupertino equivalent of Material [ListTile].
/// It comes in two forms, an old-fashioned edge-to-edge variant known from iOS
/// Settings app and in a new, "Inset Grouped" form, known from either iOS Notes
/// or Reminders app. The first is constructed using default constructor, and
/// the latter using named constructor [CupertinoListTile.notched].
///
/// The [title], [subtitle], and [additionalInfo] are usually [Text] widgets.
/// They are all limited to one line so it is a responsibility of the caller to
/// take care of text wrapping.
///
/// The size of [leading] is by default constrained to match the iOS size,
/// depending of the type of list tile. This can however be overridden by
/// providing [leadingSize]. The [trailing] widget is not constrained and is
/// therefore a responsibility of the caller to ensure reasonable size of the
/// [trailing] widget.
///
/// The background color of the tile can be set with [backgroundColor] for the
/// state before tile was tapped and with [backgroundColorActivated] for the
/// state after the tile was tapped. By default, both values are set to match
/// the default iOS appearance.
///
/// The [padding] and [leadingToTitle] are by default set to match iOS but can
/// be overwritten if necessary.
///
/// The [onTap] callback provides an option to react to taps anywhere inside the
/// list tile. This can be used to navigate routes and according to iOS
/// behavior it should not be used for example to toggle the [CupertinoSwitch]
/// in the trailing widget.
///
/// See also:
///
/// * [CupertinoListSection], an iOS-style list that is a typical container for
/// [CupertinoListTile].
/// * [ListTile], a Material Design list tile.
class CupertinoListTile extends StatefulWidget {
/// Creates an edge-to-edge iOS-style list tile like the tiles in iOS Settings
/// app.
///
/// The [title] parameter is required. It is used to convey the most important
/// information of list tile. It is typically a [Text].
///
/// The [subtitle] parameter is used to display additional information. It is
/// placed below the [title].
///
/// The [additionalInfo] parameter is used to display additional information.
/// It is placed at the end of the tile, before the [trailing] if supplied.
///
/// The [leading] parameter is typically an [Icon] or an [Image] and it comes
/// at the start of the tile. If omitted in all list tiles, a `hasLeading` of
/// enclosing [CupertinoListSection] should be set to `false` to ensure
/// correct margin of divider between tiles.
///
/// The [trailing] parameter is typically a [CupertinoListTileChevron], an
/// [Icon], or a [CupertinoButton]. It is placed at the very end of the tile.
///
/// The [onTap] parameter is used to provide an action that is called when the
/// tile is tapped. It is mainly used for navigating to a new route. It should
/// not be used to toggle a trailing [CupertinoSwitch] and similar use cases
/// because when tile is tapped, it switches the background color and remains
/// changed. This is according to iOS behavior.
///
/// The [backgroundColor] provides a custom background color for the tile in
/// a state before tapped. By default, it matches the theme's background color
/// which is by default a [CupertinoColors.systemBackground].
///
/// The [backgroundColorActivated] provides a custom background color for the
/// tile after it was tapped. By default, it matches the theme's background
/// color which is by default a [CupertinoColors.systemGrey4].
///
/// The [padding] parameter sets the padding of the content inside the tile.
/// It defaults to a value that matches the iOS look, depending on a type of
/// [CupertinoListTile]. For native look, it should not be provided.
///
/// The [leadingSize] constrains the width and height of the leading widget.
/// By default, it is set to a value that matches the iOS look, depending on a
/// type of [CupertinoListTile]. For native look, it should not be provided.
///
/// The [leadingToTitle] specifies the horizontal space between [leading] and
/// [title] widgets. By default, it is set to a value that matched the iOS
/// look, depending on a type of [CupertinoListTile]. For native look, it
/// should not be provided.
const CupertinoListTile({
super.key,
required this.title,
this.subtitle,
this.additionalInfo,
this.leading,
this.trailing,
this.onTap,
this.backgroundColor,
this.backgroundColorActivated,
this.padding,
this.leadingSize = _kLeadingSize,
this.leadingToTitle = _kLeadingToTitle,
}) : _type = _CupertinoListTileType.base;
/// Creates a notched iOS-style list tile like the tiles in iOS Notes app or
/// Reminders app.
///
/// The [title] parameter is required. It is used to convey the most important
/// information of list tile. It is typically a [Text].
///
/// The [subtitle] parameter is used to display additional information. It is
/// placed below the [title].
///
/// The [additionalInfo] parameter is used to display additional information.
/// It is placed at the end of the tile, before the [trailing] if supplied.
///
/// The [leading] parameter is typically an [Icon] or an [Image] and it comes
/// at the start of the tile. If omitted in all list tiles, a `hasLeading` of
/// enclosing [CupertinoListSection] should be set to `false` to ensure
/// correct margin of divider between tiles. For Notes-like tile appearance,
/// the [leading] can be left `null`.
///
/// The [trailing] parameter is typically a [CupertinoListTileChevron], an
/// [Icon], or a [CupertinoButton]. It is placed at the very end of the tile.
/// For Notes-like tile appearance, the [trailing] can be left `null`.
///
/// The [onTap] parameter is used to provide an action that is called when the
/// tile is tapped. It is mainly used for navigating to a new route. It should
/// not be used to toggle a trailing [CupertinoSwitch] and similar use cases
/// because when tile is tapped, it switches the background color and remains
/// changed. This is according to iOS behavior.
///
/// The [backgroundColor] provides a custom background color for the tile in
/// a state before tapped. By default, it matches the theme's background color
/// which is by default a [CupertinoColors.systemBackground].
///
/// The [backgroundColorActivated] provides a custom background color for the
/// tile after it was tapped. By default, it matches the theme's background
/// color which is by default a [CupertinoColors.systemGrey4].
///
/// The [padding] parameter sets the padding of the content inside the tile.
/// It defaults to a value that matches the iOS look, depending on a type of
/// [CupertinoListTile]. For native look, it should not be provided.
///
/// The [leadingSize] constrains the width and height of the leading widget.
/// By default, it is set to a value that matches the iOS look, depending on a
/// type of [CupertinoListTile]. For native look, it should not be provided.
///
/// The [leadingToTitle] specifies the horizontal space between [leading] and
/// [title] widgets. By default, it is set to a value that matched the iOS
/// look, depending on a type of [CupertinoListTile]. For native look, it
/// should not be provided.
const CupertinoListTile.notched({
super.key,
required this.title,
this.subtitle,
this.additionalInfo,
this.leading,
this.trailing,
this.onTap,
this.backgroundColor,
this.backgroundColorActivated,
this.padding,
this.leadingSize = _kNotchedLeadingSize,
this.leadingToTitle = _kNotchedLeadingToTitle,
}) : _type = _CupertinoListTileType.notched;
final _CupertinoListTileType _type;
/// A [title] is used to convey the central information. Usually a [Text].
final Widget title;
/// A [subtitle] is used to display additional information. It is located
/// below [title]. Usually a [Text] widget.
final Widget? subtitle;
/// Similar to [subtitle], an [additionalInfo] is used to display additional
/// information. However, instead of being displayed below [title], it is
/// displayed on the right, before [trailing]. Usually a [Text] widget.
final Widget? additionalInfo;
/// A widget displayed at the start of the [CupertinoListTile]. This is
/// typically an `Icon` or an `Image`.
final Widget? leading;
/// A widget displayed at the end of the [CupertinoListTile]. This is usually
/// a right chevron icon (e.g. `CupertinoListTileChevron`), or an `Icon`.
final Widget? trailing;
/// The [onTap] function is called when a user taps on [CupertinoListTile]. If
/// left `null`, the [CupertinoListTile] will not react on taps. If this is a
/// `Future<void> Function()`, then the [CupertinoListTile] remains activated
/// until the returned future is awaited. This is according to iOS behavior.
/// However, if this function is a `void Function()`, then the tile is active
/// only for the duration of invocation.
final FutureOr<void> Function()? onTap;
/// The [backgroundColor] of the tile in normal state. Once the tile is
/// tapped, the background color switches to [backgroundColorActivated]. It is
/// set to match the iOS look by default.
final Color? backgroundColor;
/// The [backgroundColorActivated] is the background color of the tile after
/// the tile was tapped. It is set to match the iOS look by default.
final Color? backgroundColorActivated;
/// Padding of the content inside [CupertinoListTile].
final EdgeInsetsGeometry? padding;
/// The [leadingSize] is used to constrain the width and height of [leading]
/// widget.
final double leadingSize;
/// The horizontal space between [leading] widget and [title].
final double leadingToTitle;
@override
State<CupertinoListTile> createState() => _CupertinoListTileState();
}
class _CupertinoListTileState extends State<CupertinoListTile> {
bool _tapped = false;
@override
Widget build(BuildContext context) {
final TextStyle titleTextStyle =
widget._type == _CupertinoListTileType.base || widget.subtitle == null
? CupertinoTheme.of(context).textTheme.textStyle
: CupertinoTheme.of(context).textTheme.textStyle.merge(
TextStyle(
fontWeight: FontWeight.w600,
fontSize: widget.leading == null ? _kNotchedTitleWithSubtitleFontSize : null,
),
);
final TextStyle subtitleTextStyle = widget._type == _CupertinoListTileType.base
? CupertinoTheme.of(context).textTheme.textStyle.merge(
TextStyle(
fontSize: _kSubtitleFontSize,
color: CupertinoColors.secondaryLabel.resolveFrom(context),
),
)
: CupertinoTheme.of(context).textTheme.textStyle.merge(
TextStyle(
fontSize: _kNotchedSubtitleFontSize,
color: CupertinoColors.secondaryLabel.resolveFrom(context),
),
);
final TextStyle? additionalInfoTextStyle = widget.additionalInfo != null
? CupertinoTheme.of(context).textTheme.textStyle.merge(
TextStyle(color: CupertinoColors.secondaryLabel.resolveFrom(context)))
: null;
final Widget title = DefaultTextStyle(
style: titleTextStyle,
maxLines: 1,
overflow: TextOverflow.ellipsis,
child: widget.title,
);
final EdgeInsetsGeometry padding = widget.padding ?? switch (widget._type) {
_CupertinoListTileType.base when widget.subtitle != null => _kPaddingWithSubtitle,
_CupertinoListTileType.notched when widget.leading != null => _kNotchedPadding,
_CupertinoListTileType.base => _kPadding,
_CupertinoListTileType.notched => _kNotchedPaddingWithoutLeading,
};
Widget? subtitle;
if (widget.subtitle != null) {
subtitle = DefaultTextStyle(
style: subtitleTextStyle,
maxLines: 1,
overflow: TextOverflow.ellipsis,
child: widget.subtitle!,
);
}
Widget? additionalInfo;
if (widget.additionalInfo != null) {
additionalInfo = DefaultTextStyle(
style: additionalInfoTextStyle!,
maxLines: 1,
child: widget.additionalInfo!,
);
}
// The color for default state tile is set to either what user provided or
// null and it will resolve to the correct color provided by context. But if
// the tile was tapped, it is set to what user provided or if null to the
// default color that matched the iOS-style.
Color? backgroundColor = widget.backgroundColor;
if (_tapped) {
backgroundColor = widget.backgroundColorActivated ?? CupertinoColors.systemGrey4.resolveFrom(context);
}
final double minHeight = switch (widget._type) {
_CupertinoListTileType.base when subtitle != null => _kMinHeightWithSubtitle,
_CupertinoListTileType.notched when widget.leading != null => _kNotchedMinHeight,
_CupertinoListTileType.base => _kMinHeight,
_CupertinoListTileType.notched => _kNotchedMinHeightWithoutLeading,
};
final Widget child = Container(
constraints: BoxConstraints(minWidth: double.infinity, minHeight: minHeight),
color: backgroundColor,
child: Padding(
padding: padding,
child: Row(
children: <Widget>[
if (widget.leading != null) ...<Widget>[
SizedBox(
width: widget.leadingSize,
height: widget.leadingSize,
child: Center(
child: widget.leading,
),
),
SizedBox(width: widget.leadingToTitle),
] else
SizedBox(height: widget.leadingSize),
Expanded(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
title,
if (subtitle != null) ...<Widget>[
const SizedBox(height: _kNotchedTitleToSubtitle),
subtitle,
],
],
),
),
if (additionalInfo != null) ...<Widget>[
additionalInfo,
if (widget.trailing != null)
const SizedBox(width: _kAdditionalInfoToTrailing),
],
if (widget.trailing != null) widget.trailing!
],
),
),
);
if (widget.onTap == null) {
return child;
}
return GestureDetector(
onTapDown: (_) => setState(() { _tapped = true; }),
onTapCancel: () => setState(() { _tapped = false; }),
onTap: () async {
await widget.onTap!();
if (mounted) {
setState(() { _tapped = false; });
}
},
behavior: HitTestBehavior.opaque,
child: child,
);
}
}
/// A typical iOS trailing widget used to denote that a `CupertinoListTile` is a
/// button with an action.
///
/// The [CupertinoListTileChevron] is meant as a convenience implementation of
/// trailing right chevron.
class CupertinoListTileChevron extends StatelessWidget {
/// Creates a typical widget used to denote that a `CupertinoListTile` is a
/// button with action.
const CupertinoListTileChevron({super.key});
@override
Widget build(BuildContext context) {
return Icon(
CupertinoIcons.right_chevron,
size: CupertinoTheme.of(context).textTheme.textStyle.fontSize,
color: CupertinoColors.systemGrey2.resolveFrom(context),
);
}
}
| flutter/packages/flutter/lib/src/cupertino/list_tile.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/list_tile.dart",
"repo_id": "flutter",
"token_count": 5781
} | 641 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'bottom_tab_bar.dart';
import 'colors.dart';
import 'theme.dart';
/// Coordinates tab selection between a [CupertinoTabBar] and a [CupertinoTabScaffold].
///
/// The [index] property is the index of the selected tab. Changing its value
/// updates the actively displayed tab of the [CupertinoTabScaffold] the
/// [CupertinoTabController] controls, as well as the currently selected tab item of
/// its [CupertinoTabBar].
///
/// {@tool snippet}
/// This samples shows how [CupertinoTabController] can be used to switch tabs in
/// [CupertinoTabScaffold].
///
/// ** See code in examples/api/lib/cupertino/tab_scaffold/cupertino_tab_controller.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [CupertinoTabScaffold], a tabbed application root layout that can be
/// controlled by a [CupertinoTabController].
/// * [RestorableCupertinoTabController], which is a restorable version
/// of this controller.
class CupertinoTabController extends ChangeNotifier {
/// Creates a [CupertinoTabController] to control the tab index of [CupertinoTabScaffold]
/// and [CupertinoTabBar].
///
/// The [initialIndex] defaults to 0. The value must be greater than or equal
/// to 0, and less than the total number of tabs.
CupertinoTabController({ int initialIndex = 0 })
: _index = initialIndex,
assert(initialIndex >= 0);
bool _isDisposed = false;
/// The index of the currently selected tab.
///
/// Changing the value of [index] updates the actively displayed tab of the
/// [CupertinoTabScaffold] controlled by this [CupertinoTabController], as well
/// as the currently selected tab item of its [CupertinoTabScaffold.tabBar].
///
/// The value must be greater than or equal to 0, and less than the total
/// number of tabs.
int get index => _index;
int _index;
set index(int value) {
assert(value >= 0);
if (_index == value) {
return;
}
_index = value;
notifyListeners();
}
@mustCallSuper
@override
void dispose() {
super.dispose();
_isDisposed = true;
}
}
/// Implements a tabbed iOS application's root layout and behavior structure.
///
/// The scaffold lays out the tab bar at the bottom and the content between or
/// behind the tab bar.
///
/// A [tabBar] and a [tabBuilder] are required. The [CupertinoTabScaffold]
/// will automatically listen to the provided [CupertinoTabBar]'s tap callbacks
/// to change the active tab.
///
/// A [controller] can be used to provide an initially selected tab index and manage
/// subsequent tab changes. If a controller is not specified, the scaffold will
/// create its own [CupertinoTabController] and manage it internally. Otherwise
/// it's up to the owner of [controller] to call `dispose` on it after finish
/// using it.
///
/// Tabs' contents are built with the provided [tabBuilder] at the active
/// tab index. The [tabBuilder] must be able to build the same number of
/// pages as there are [tabBar] items. Inactive tabs will be moved [Offstage]
/// and their animations disabled.
///
/// Adding/removing tabs, or changing the order of tabs is supported but not
/// recommended. Doing so is against the iOS human interface guidelines, and
/// [CupertinoTabScaffold] may lose some tabs' state in the process.
///
/// Use [CupertinoTabView] as the root widget of each tab to support tabs with
/// parallel navigation state and history. Since each [CupertinoTabView] contains
/// a [Navigator], rebuilding the [CupertinoTabView] with a different
/// [WidgetBuilder] instance in [CupertinoTabView.builder] will not recreate
/// the [CupertinoTabView]'s navigation stack or update its UI. To update the
/// contents of the [CupertinoTabView] after it's built, trigger a rebuild
/// (via [State.setState], for instance) from its descendant rather than from
/// its ancestor.
///
/// {@tool dartpad}
/// A sample code implementing a typical iOS information architecture with tabs.
///
/// ** See code in examples/api/lib/cupertino/tab_scaffold/cupertino_tab_scaffold.0.dart **
/// {@end-tool}
///
/// To push a route above all tabs instead of inside the currently selected one
/// (such as when showing a dialog on top of this scaffold), use
/// `Navigator.of(rootNavigator: true)` from inside the [BuildContext] of a
/// [CupertinoTabView].
///
/// See also:
///
/// * [CupertinoTabBar], the bottom tab bar inserted in the scaffold.
/// * [CupertinoTabController], the selection state of this widget.
/// * [CupertinoTabView], the typical root content of each tab that holds its own
/// [Navigator] stack.
/// * [CupertinoPageRoute], a route hosting modal pages with iOS style transitions.
/// * [CupertinoPageScaffold], typical contents of an iOS modal page implementing
/// layout with a navigation bar on top.
/// * [iOS human interface guidelines](https://developer.apple.com/design/human-interface-guidelines/ios/bars/tab-bars/).
class CupertinoTabScaffold extends StatefulWidget {
/// Creates a layout for applications with a tab bar at the bottom.
CupertinoTabScaffold({
super.key,
required this.tabBar,
required this.tabBuilder,
this.controller,
this.backgroundColor,
this.resizeToAvoidBottomInset = true,
this.restorationId,
}) : assert(
controller == null || controller.index < tabBar.items.length,
"The CupertinoTabController's current index ${controller.index} is "
'out of bounds for the tab bar with ${tabBar.items.length} tabs',
);
/// The [tabBar] is a [CupertinoTabBar] drawn at the bottom of the screen
/// that lets the user switch between different tabs in the main content area
/// when present.
///
/// The [CupertinoTabBar.currentIndex] is only used to initialize a
/// [CupertinoTabController] when no [controller] is provided. Subsequently
/// providing a different [CupertinoTabBar.currentIndex] does not affect the
/// scaffold or the tab bar's active tab index. To programmatically change
/// the active tab index, use a [CupertinoTabController].
///
/// If [CupertinoTabBar.onTap] is provided, it will still be called.
/// [CupertinoTabScaffold] automatically also listen to the
/// [CupertinoTabBar]'s `onTap` to change the [controller]'s `index`
/// and change the actively displayed tab in [CupertinoTabScaffold]'s own
/// main content area.
///
/// If translucent, the main content may slide behind it.
/// Otherwise, the main content's bottom margin will be offset by its height.
///
/// By default [tabBar] disables text scaling to match the native iOS behavior.
/// To override this behavior, wrap each of the [tabBar]'s items inside a
/// [MediaQuery] with the desired [TextScaler].
final CupertinoTabBar tabBar;
/// Controls the currently selected tab index of the [tabBar], as well as the
/// active tab index of the [tabBuilder]. Providing a different [controller]
/// will also update the scaffold's current active index to the new controller's
/// index value.
///
/// Defaults to null.
final CupertinoTabController? controller;
/// An [IndexedWidgetBuilder] that's called when tabs become active.
///
/// The widgets built by [IndexedWidgetBuilder] are typically a
/// [CupertinoTabView] in order to achieve the parallel hierarchical
/// information architecture seen on iOS apps with tab bars.
///
/// When the tab becomes inactive, its content is cached in the widget tree
/// [Offstage] and its animations disabled.
///
/// Content can slide under the [tabBar] when they're translucent.
/// In that case, the child's [BuildContext]'s [MediaQuery] will have a
/// bottom padding indicating the area of obstructing overlap from the
/// [tabBar].
final IndexedWidgetBuilder tabBuilder;
/// The color of the widget that underlies the entire scaffold.
///
/// By default uses [CupertinoTheme]'s `scaffoldBackgroundColor` when null.
final Color? backgroundColor;
/// Whether the body should size itself to avoid the window's bottom inset.
///
/// For example, if there is an onscreen keyboard displayed above the
/// scaffold, the body can be resized to avoid overlapping the keyboard, which
/// prevents widgets inside the body from being obscured by the keyboard.
///
/// Defaults to true.
final bool resizeToAvoidBottomInset;
/// Restoration ID to save and restore the state of the [CupertinoTabScaffold].
///
/// This property only has an effect when no [controller] has been provided:
/// If it is non-null (and no [controller] has been provided), the scaffold
/// will persist and restore the currently selected tab index. If a
/// [controller] has been provided, it is the responsibility of the owner of
/// that controller to persist and restore it, e.g. by using a
/// [RestorableCupertinoTabController].
///
/// The state of this widget is persisted in a [RestorationBucket] claimed
/// from the surrounding [RestorationScope] using the provided restoration ID.
///
/// See also:
///
/// * [RestorationManager], which explains how state restoration works in
/// Flutter.
final String? restorationId;
@override
State<CupertinoTabScaffold> createState() => _CupertinoTabScaffoldState();
}
class _CupertinoTabScaffoldState extends State<CupertinoTabScaffold> with RestorationMixin {
RestorableCupertinoTabController? _internalController;
CupertinoTabController get _controller => widget.controller ?? _internalController!.value;
@override
String? get restorationId => widget.restorationId;
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
_restoreInternalController();
}
void _restoreInternalController() {
if (_internalController != null) {
registerForRestoration(_internalController!, 'controller');
_internalController!.value.addListener(_onCurrentIndexChange);
}
}
@override
void initState() {
super.initState();
_updateTabController();
}
void _updateTabController([CupertinoTabController? oldWidgetController]) {
if (widget.controller == null && _internalController == null) {
// No widget-provided controller: create an internal controller.
_internalController = RestorableCupertinoTabController(initialIndex: widget.tabBar.currentIndex);
if (!restorePending) {
_restoreInternalController(); // Also adds the listener to the controller.
}
}
if (widget.controller != null && _internalController != null) {
// Use the widget-provided controller.
unregisterFromRestoration(_internalController!);
_internalController!.dispose();
_internalController = null;
}
if (oldWidgetController != widget.controller) {
// The widget-provided controller has changed: move listeners.
if (oldWidgetController?._isDisposed == false) {
oldWidgetController!.removeListener(_onCurrentIndexChange);
}
widget.controller?.addListener(_onCurrentIndexChange);
}
}
void _onCurrentIndexChange() {
assert(
_controller.index >= 0 && _controller.index < widget.tabBar.items.length,
"The $runtimeType's current index ${_controller.index} is "
'out of bounds for the tab bar with ${widget.tabBar.items.length} tabs',
);
// The value of `_controller.index` has already been updated at this point.
// Calling `setState` to rebuild using `_controller.index`.
setState(() {});
}
@override
void didUpdateWidget(CupertinoTabScaffold oldWidget) {
super.didUpdateWidget(oldWidget);
if (widget.controller != oldWidget.controller) {
_updateTabController(oldWidget.controller);
} else if (_controller.index >= widget.tabBar.items.length) {
// If a new [tabBar] with less than (_controller.index + 1) items is provided,
// clamp the current index.
_controller.index = widget.tabBar.items.length - 1;
}
}
@override
Widget build(BuildContext context) {
final MediaQueryData existingMediaQuery = MediaQuery.of(context);
MediaQueryData newMediaQuery = MediaQuery.of(context);
Widget content = _TabSwitchingView(
currentTabIndex: _controller.index,
tabCount: widget.tabBar.items.length,
tabBuilder: widget.tabBuilder,
);
EdgeInsets contentPadding = EdgeInsets.zero;
if (widget.resizeToAvoidBottomInset) {
// Remove the view inset and add it back as a padding in the inner content.
newMediaQuery = newMediaQuery.removeViewInsets(removeBottom: true);
contentPadding = EdgeInsets.only(bottom: existingMediaQuery.viewInsets.bottom);
}
// Only pad the content with the height of the tab bar if the tab
// isn't already entirely obstructed by a keyboard or other view insets.
// Don't double pad.
if (!widget.resizeToAvoidBottomInset || widget.tabBar.preferredSize.height > existingMediaQuery.viewInsets.bottom) {
// TODO(xster): Use real size after partial layout instead of preferred size.
// https://github.com/flutter/flutter/issues/12912
final double bottomPadding =
widget.tabBar.preferredSize.height + existingMediaQuery.padding.bottom;
// If tab bar opaque, directly stop the main content higher. If
// translucent, let main content draw behind the tab bar but hint the
// obstructed area.
if (widget.tabBar.opaque(context)) {
contentPadding = EdgeInsets.only(bottom: bottomPadding);
newMediaQuery = newMediaQuery.removePadding(removeBottom: true);
} else {
newMediaQuery = newMediaQuery.copyWith(
padding: newMediaQuery.padding.copyWith(
bottom: bottomPadding,
),
);
}
}
content = MediaQuery(
data: newMediaQuery,
child: Padding(
padding: contentPadding,
child: content,
),
);
return DecoratedBox(
decoration: BoxDecoration(
color: CupertinoDynamicColor.maybeResolve(widget.backgroundColor, context)
?? CupertinoTheme.of(context).scaffoldBackgroundColor,
),
child: Stack(
children: <Widget>[
// The main content being at the bottom is added to the stack first.
content,
MediaQuery.withNoTextScaling(
child: Align(
alignment: Alignment.bottomCenter,
// Override the tab bar's currentIndex to the current tab and hook in
// our own listener to update the [_controller.currentIndex] on top of a possibly user
// provided callback.
child: widget.tabBar.copyWith(
currentIndex: _controller.index,
onTap: (int newIndex) {
_controller.index = newIndex;
// Chain the user's original callback.
widget.tabBar.onTap?.call(newIndex);
},
),
),
),
],
),
);
}
@override
void dispose() {
if (widget.controller?._isDisposed == false) {
_controller.removeListener(_onCurrentIndexChange);
}
_internalController?.dispose();
super.dispose();
}
}
/// A widget laying out multiple tabs with only one active tab being built
/// at a time and on stage. Off stage tabs' animations are stopped.
class _TabSwitchingView extends StatefulWidget {
const _TabSwitchingView({
required this.currentTabIndex,
required this.tabCount,
required this.tabBuilder,
}) : assert(tabCount > 0);
final int currentTabIndex;
final int tabCount;
final IndexedWidgetBuilder tabBuilder;
@override
_TabSwitchingViewState createState() => _TabSwitchingViewState();
}
class _TabSwitchingViewState extends State<_TabSwitchingView> {
final List<bool> shouldBuildTab = <bool>[];
final List<FocusScopeNode> tabFocusNodes = <FocusScopeNode>[];
// When focus nodes are no longer needed, we need to dispose of them, but we
// can't be sure that nothing else is listening to them until this widget is
// disposed of, so when they are no longer needed, we move them to this list,
// and dispose of them when we dispose of this widget.
final List<FocusScopeNode> discardedNodes = <FocusScopeNode>[];
@override
void initState() {
super.initState();
shouldBuildTab.addAll(List<bool>.filled(widget.tabCount, false));
}
@override
void didChangeDependencies() {
super.didChangeDependencies();
_focusActiveTab();
}
@override
void didUpdateWidget(_TabSwitchingView oldWidget) {
super.didUpdateWidget(oldWidget);
// Only partially invalidate the tabs cache to avoid breaking the current
// behavior. We assume that the only possible change is either:
// - new tabs are appended to the tab list, or
// - some trailing tabs are removed.
// If the above assumption is not true, some tabs may lose their state.
final int lengthDiff = widget.tabCount - shouldBuildTab.length;
if (lengthDiff > 0) {
shouldBuildTab.addAll(List<bool>.filled(lengthDiff, false));
} else if (lengthDiff < 0) {
shouldBuildTab.removeRange(widget.tabCount, shouldBuildTab.length);
}
_focusActiveTab();
}
// Will focus the active tab if the FocusScope above it has focus already. If
// not, then it will just mark it as the preferred focus for that scope.
void _focusActiveTab() {
if (tabFocusNodes.length != widget.tabCount) {
if (tabFocusNodes.length > widget.tabCount) {
discardedNodes.addAll(tabFocusNodes.sublist(widget.tabCount));
tabFocusNodes.removeRange(widget.tabCount, tabFocusNodes.length);
} else {
tabFocusNodes.addAll(
List<FocusScopeNode>.generate(
widget.tabCount - tabFocusNodes.length,
(int index) => FocusScopeNode(debugLabel: '$CupertinoTabScaffold Tab ${index + tabFocusNodes.length}'),
),
);
}
}
FocusScope.of(context).setFirstFocus(tabFocusNodes[widget.currentTabIndex]);
}
@override
void dispose() {
for (final FocusScopeNode focusScopeNode in tabFocusNodes) {
focusScopeNode.dispose();
}
for (final FocusScopeNode focusScopeNode in discardedNodes) {
focusScopeNode.dispose();
}
super.dispose();
}
@override
Widget build(BuildContext context) {
return Stack(
fit: StackFit.expand,
children: List<Widget>.generate(widget.tabCount, (int index) {
final bool active = index == widget.currentTabIndex;
shouldBuildTab[index] = active || shouldBuildTab[index];
return HeroMode(
enabled: active,
child: Offstage(
offstage: !active,
child: TickerMode(
enabled: active,
child: FocusScope(
node: tabFocusNodes[index],
child: Builder(builder: (BuildContext context) {
return shouldBuildTab[index] ? widget.tabBuilder(context, index) : const SizedBox.shrink();
}),
),
),
),
);
}),
);
}
}
/// A [RestorableProperty] that knows how to store and restore a
/// [CupertinoTabController].
///
/// The [CupertinoTabController] is accessible via the [value] getter. During
/// state restoration, the property will restore [CupertinoTabController.index]
/// to the value it had when the restoration data it is getting restored from
/// was collected.
class RestorableCupertinoTabController extends RestorableChangeNotifier<CupertinoTabController> {
/// Creates a [RestorableCupertinoTabController] to control the tab index of
/// [CupertinoTabScaffold] and [CupertinoTabBar].
///
/// The `initialIndex` defaults to zero. The value must be greater than or
/// equal to zero, and less than the total number of tabs.
RestorableCupertinoTabController({ int initialIndex = 0 })
: assert(initialIndex >= 0),
_initialIndex = initialIndex;
final int _initialIndex;
@override
CupertinoTabController createDefaultValue() {
return CupertinoTabController(initialIndex: _initialIndex);
}
@override
CupertinoTabController fromPrimitives(Object? data) {
assert(data != null);
return CupertinoTabController(initialIndex: data! as int);
}
@override
Object? toPrimitives() {
return value.index;
}
}
| flutter/packages/flutter/lib/src/cupertino/tab_scaffold.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/cupertino/tab_scaffold.dart",
"repo_id": "flutter",
"token_count": 6593
} | 642 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:js_interop';
// This value is set by the engine. It is used to determine if the application is
// using canvaskit.
@JS('window.flutterCanvasKit')
external JSAny? get _windowFlutterCanvasKit;
/// The web implementation of [isCanvasKit]
bool get isCanvasKit => _windowFlutterCanvasKit != null;
| flutter/packages/flutter/lib/src/foundation/_capabilities_web.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/foundation/_capabilities_web.dart",
"repo_id": "flutter",
"token_count": 137
} | 643 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
/// A constant that is true if the application was compiled in release mode.
///
/// More specifically, this is a constant that is true if the application was
/// compiled in Dart with the '-Ddart.vm.product=true' flag.
///
/// Since this is a const value, it can be used to indicate to the compiler that
/// a particular block of code will not be executed in release mode, and hence
/// can be removed.
///
/// Generally it is better to use [kDebugMode] or `assert` to gate code, since
/// using [kReleaseMode] will introduce differences between release and profile
/// builds, which makes performance testing less representative.
///
/// See also:
///
/// * [kDebugMode], which is true in debug builds.
/// * [kProfileMode], which is true in profile builds.
const bool kReleaseMode = bool.fromEnvironment('dart.vm.product');
/// A constant that is true if the application was compiled in profile mode.
///
/// More specifically, this is a constant that is true if the application was
/// compiled in Dart with the '-Ddart.vm.profile=true' flag.
///
/// Since this is a const value, it can be used to indicate to the compiler that
/// a particular block of code will not be executed in profile mode, an hence
/// can be removed.
///
/// See also:
///
/// * [kDebugMode], which is true in debug builds.
/// * [kReleaseMode], which is true in release builds.
const bool kProfileMode = bool.fromEnvironment('dart.vm.profile');
/// A constant that is true if the application was compiled in debug mode.
///
/// More specifically, this is a constant that is true if the application was
/// not compiled with '-Ddart.vm.product=true' and '-Ddart.vm.profile=true'.
///
/// Since this is a const value, it can be used to indicate to the compiler that
/// a particular block of code will not be executed in debug mode, and hence
/// can be removed.
///
/// An alternative strategy is to use asserts, as in:
///
/// ```dart
/// assert(() {
/// // ...debug-only code here...
/// return true;
/// }());
/// ```
///
/// See also:
///
/// * [kReleaseMode], which is true in release builds.
/// * [kProfileMode], which is true in profile builds.
const bool kDebugMode = !kReleaseMode && !kProfileMode;
/// The epsilon of tolerable double precision error.
///
/// This is used in various places in the framework to allow for floating point
/// precision loss in calculations. Differences below this threshold are safe to
/// disregard.
const double precisionErrorTolerance = 1e-10;
/// A constant that is true if the application was compiled to run on the web.
///
/// See also:
///
/// * [defaultTargetPlatform], which is used by themes to find out which
/// platform the application is running on (or, in the case of a web app,
/// which platform the application's browser is running in). Can be overridden
/// in tests with [debugDefaultTargetPlatformOverride].
/// * [dart:io.Platform], a way to find out the browser's platform that is not
/// overridable in tests.
const bool kIsWeb = bool.fromEnvironment('dart.library.js_util');
| flutter/packages/flutter/lib/src/foundation/constants.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/foundation/constants.dart",
"repo_id": "flutter",
"token_count": 849
} | 644 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
/// A [Future] whose [then] implementation calls the callback immediately.
///
/// This is similar to [Future.value], except that the value is available in
/// the same event-loop iteration.
///
/// ⚠ This class is useful in cases where you want to expose a single API, where
/// you normally want to have everything execute synchronously, but where on
/// rare occasions you want the ability to switch to an asynchronous model. **In
/// general use of this class should be avoided as it is very difficult to debug
/// such bimodal behavior.**
///
/// A [SynchronousFuture] will never complete with an error.
class SynchronousFuture<T> implements Future<T> {
/// Creates a synchronous future.
///
/// See also:
///
/// * [Future.value] for information about creating a regular
/// [Future] that completes with a value.
SynchronousFuture(this._value);
final T _value;
@override
Stream<T> asStream() {
final StreamController<T> controller = StreamController<T>();
controller.add(_value);
controller.close();
return controller.stream;
}
@override
Future<T> catchError(Function onError, { bool Function(Object error)? test }) => Completer<T>().future;
@override
Future<R> then<R>(FutureOr<R> Function(T value) onValue, { Function? onError }) {
final FutureOr<R> result = onValue(_value);
if (result is Future<R>) {
return result;
}
return SynchronousFuture<R>(result);
}
@override
Future<T> timeout(Duration timeLimit, { FutureOr<T> Function()? onTimeout }) {
return Future<T>.value(_value).timeout(timeLimit, onTimeout: onTimeout);
}
@override
Future<T> whenComplete(FutureOr<dynamic> Function() action) {
try {
final FutureOr<dynamic> result = action();
if (result is Future) {
return result.then<T>((dynamic value) => _value);
}
return this;
} catch (e, stack) {
return Future<T>.error(e, stack);
}
}
}
| flutter/packages/flutter/lib/src/foundation/synchronous_future.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/foundation/synchronous_future.dart",
"repo_id": "flutter",
"token_count": 675
} | 645 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
// TODO(abarth): Consider using vector_math.
class _Vector {
_Vector(int size)
: _offset = 0,
_length = size,
_elements = Float64List(size);
_Vector.fromVOL(List<double> values, int offset, int length)
: _offset = offset,
_length = length,
_elements = values;
final int _offset;
final int _length;
final List<double> _elements;
double operator [](int i) => _elements[i + _offset];
void operator []=(int i, double value) {
_elements[i + _offset] = value;
}
double operator *(_Vector a) {
double result = 0.0;
for (int i = 0; i < _length; i += 1) {
result += this[i] * a[i];
}
return result;
}
double norm() => math.sqrt(this * this);
}
// TODO(abarth): Consider using vector_math.
class _Matrix {
_Matrix(int rows, int cols)
: _columns = cols,
_elements = Float64List(rows * cols);
final int _columns;
final List<double> _elements;
double get(int row, int col) => _elements[row * _columns + col];
void set(int row, int col, double value) {
_elements[row * _columns + col] = value;
}
_Vector getRow(int row) => _Vector.fromVOL(
_elements,
row * _columns,
_columns,
);
}
/// An nth degree polynomial fit to a dataset.
class PolynomialFit {
/// Creates a polynomial fit of the given degree.
///
/// There are n + 1 coefficients in a fit of degree n.
PolynomialFit(int degree) : coefficients = Float64List(degree + 1);
/// The polynomial coefficients of the fit.
///
/// For each `i`, the element `coefficients[i]` is the coefficient of
/// the `i`-th power of the variable.
final List<double> coefficients;
/// An indicator of the quality of the fit.
///
/// Larger values indicate greater quality. The value ranges from 0.0 to 1.0.
///
/// The confidence is defined as the fraction of the dataset's variance
/// that is captured by variance in the fit polynomial. In statistics
/// textbooks this is often called "r-squared".
late double confidence;
@override
String toString() {
final String coefficientString =
coefficients.map((double c) => c.toStringAsPrecision(3)).toList().toString();
return '${objectRuntimeType(this, 'PolynomialFit')}($coefficientString, confidence: ${confidence.toStringAsFixed(3)})';
}
}
/// Uses the least-squares algorithm to fit a polynomial to a set of data.
class LeastSquaresSolver {
/// Creates a least-squares solver.
LeastSquaresSolver(this.x, this.y, this.w)
: assert(x.length == y.length),
assert(y.length == w.length);
/// The x-coordinates of each data point.
final List<double> x;
/// The y-coordinates of each data point.
final List<double> y;
/// The weight to use for each data point.
final List<double> w;
/// Fits a polynomial of the given degree to the data points.
///
/// When there is not enough data to fit a curve null is returned.
PolynomialFit? solve(int degree) {
if (degree > x.length) {
// Not enough data to fit a curve.
return null;
}
final PolynomialFit result = PolynomialFit(degree);
// Shorthands for the purpose of notation equivalence to original C++ code.
final int m = x.length;
final int n = degree + 1;
// Expand the X vector to a matrix A, pre-multiplied by the weights.
final _Matrix a = _Matrix(n, m);
for (int h = 0; h < m; h += 1) {
a.set(0, h, w[h]);
for (int i = 1; i < n; i += 1) {
a.set(i, h, a.get(i - 1, h) * x[h]);
}
}
// Apply the Gram-Schmidt process to A to obtain its QR decomposition.
// Orthonormal basis, column-major ordVectorer.
final _Matrix q = _Matrix(n, m);
// Upper triangular matrix, row-major order.
final _Matrix r = _Matrix(n, n);
for (int j = 0; j < n; j += 1) {
for (int h = 0; h < m; h += 1) {
q.set(j, h, a.get(j, h));
}
for (int i = 0; i < j; i += 1) {
final double dot = q.getRow(j) * q.getRow(i);
for (int h = 0; h < m; h += 1) {
q.set(j, h, q.get(j, h) - dot * q.get(i, h));
}
}
final double norm = q.getRow(j).norm();
if (norm < precisionErrorTolerance) {
// Vectors are linearly dependent or zero so no solution.
return null;
}
final double inverseNorm = 1.0 / norm;
for (int h = 0; h < m; h += 1) {
q.set(j, h, q.get(j, h) * inverseNorm);
}
for (int i = 0; i < n; i += 1) {
r.set(j, i, i < j ? 0.0 : q.getRow(j) * a.getRow(i));
}
}
// Solve R B = Qt W Y to find B. This is easy because R is upper triangular.
// We just work from bottom-right to top-left calculating B's coefficients.
final _Vector wy = _Vector(m);
for (int h = 0; h < m; h += 1) {
wy[h] = y[h] * w[h];
}
for (int i = n - 1; i >= 0; i -= 1) {
result.coefficients[i] = q.getRow(i) * wy;
for (int j = n - 1; j > i; j -= 1) {
result.coefficients[i] -= r.get(i, j) * result.coefficients[j];
}
result.coefficients[i] /= r.get(i, i);
}
// Calculate the coefficient of determination (confidence) as:
// 1 - (sumSquaredError / sumSquaredTotal)
// ...where sumSquaredError is the residual sum of squares (variance of the
// error), and sumSquaredTotal is the total sum of squares (variance of the
// data) where each has been weighted.
double yMean = 0.0;
for (int h = 0; h < m; h += 1) {
yMean += y[h];
}
yMean /= m;
double sumSquaredError = 0.0;
double sumSquaredTotal = 0.0;
for (int h = 0; h < m; h += 1) {
double term = 1.0;
double err = y[h] - result.coefficients[0];
for (int i = 1; i < n; i += 1) {
term *= x[h];
err -= term * result.coefficients[i];
}
sumSquaredError += w[h] * w[h] * err * err;
final double v = y[h] - yMean;
sumSquaredTotal += w[h] * w[h] * v * v;
}
result.confidence = sumSquaredTotal <= precisionErrorTolerance ? 1.0 :
1.0 - (sumSquaredError / sumSquaredTotal);
return result;
}
}
| flutter/packages/flutter/lib/src/gestures/lsq_solver.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/gestures/lsq_solver.dart",
"repo_id": "flutter",
"token_count": 2532
} | 646 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'action_buttons.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// A [ActionIconThemeData] that overrides the default icons of
/// [BackButton], [CloseButton], [DrawerButton], and [EndDrawerButton] with
/// [ActionIconTheme.of] or the overall [Theme]'s [ThemeData.actionIconTheme].
@immutable
class ActionIconThemeData with Diagnosticable {
/// Creates an [ActionIconThemeData].
///
/// The builders [backButtonIconBuilder], [closeButtonIconBuilder],
/// [drawerButtonIconBuilder], [endDrawerButtonIconBuilder] may be null.
const ActionIconThemeData({ this.backButtonIconBuilder, this.closeButtonIconBuilder, this.drawerButtonIconBuilder, this.endDrawerButtonIconBuilder });
/// Overrides [BackButtonIcon]'s icon.
///
/// If [backButtonIconBuilder] is null, then [BackButtonIcon]
/// fallbacks to the platform's default back button icon.
final WidgetBuilder? backButtonIconBuilder;
/// Overrides [CloseButtonIcon]'s icon.
///
/// If [closeButtonIconBuilder] is null, then [CloseButtonIcon]
/// fallbacks to the platform's default close button icon.
final WidgetBuilder? closeButtonIconBuilder;
/// Overrides [DrawerButtonIcon]'s icon.
///
/// If [drawerButtonIconBuilder] is null, then [DrawerButtonIcon]
/// fallbacks to the platform's default drawer button icon.
final WidgetBuilder? drawerButtonIconBuilder;
/// Overrides [EndDrawerButtonIcon]'s icon.
///
/// If [endDrawerButtonIconBuilder] is null, then [EndDrawerButtonIcon]
/// fallbacks to the platform's default end drawer button icon.
final WidgetBuilder? endDrawerButtonIconBuilder;
/// Creates a copy of this object but with the given fields replaced with the
/// new values.
ActionIconThemeData copyWith({
WidgetBuilder? backButtonIconBuilder,
WidgetBuilder? closeButtonIconBuilder,
WidgetBuilder? drawerButtonIconBuilder,
WidgetBuilder? endDrawerButtonIconBuilder,
}) {
return ActionIconThemeData(
backButtonIconBuilder: backButtonIconBuilder ?? this.backButtonIconBuilder,
closeButtonIconBuilder: closeButtonIconBuilder ?? this.closeButtonIconBuilder,
drawerButtonIconBuilder: drawerButtonIconBuilder ?? this.drawerButtonIconBuilder,
endDrawerButtonIconBuilder: endDrawerButtonIconBuilder ?? this.endDrawerButtonIconBuilder,
);
}
/// Linearly interpolate between two action icon themes.
static ActionIconThemeData? lerp(ActionIconThemeData? a, ActionIconThemeData? b, double t) {
if (a == null && b == null) {
return null;
}
return ActionIconThemeData(
backButtonIconBuilder: t < 0.5 ? a?.backButtonIconBuilder : b?.backButtonIconBuilder,
closeButtonIconBuilder: t < 0.5 ? a?.closeButtonIconBuilder : b?.closeButtonIconBuilder,
drawerButtonIconBuilder: t < 0.5 ? a?.drawerButtonIconBuilder : b?.drawerButtonIconBuilder,
endDrawerButtonIconBuilder: t < 0.5 ? a?.endDrawerButtonIconBuilder : b?.endDrawerButtonIconBuilder,
);
}
@override
int get hashCode {
final List<Object?> values = <Object?>[
backButtonIconBuilder,
closeButtonIconBuilder,
drawerButtonIconBuilder,
endDrawerButtonIconBuilder,
];
return Object.hashAll(values);
}
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is ActionIconThemeData
&& other.backButtonIconBuilder == backButtonIconBuilder
&& other.closeButtonIconBuilder == closeButtonIconBuilder
&& other.drawerButtonIconBuilder == drawerButtonIconBuilder
&& other.endDrawerButtonIconBuilder == endDrawerButtonIconBuilder;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<WidgetBuilder>('backButtonIconBuilder', backButtonIconBuilder, defaultValue: null));
properties.add(DiagnosticsProperty<WidgetBuilder>('closeButtonIconBuilder', closeButtonIconBuilder, defaultValue: null));
properties.add(DiagnosticsProperty<WidgetBuilder>('drawerButtonIconBuilder', drawerButtonIconBuilder, defaultValue: null));
properties.add(DiagnosticsProperty<WidgetBuilder>('endDrawerButtonIconBuilder', endDrawerButtonIconBuilder, defaultValue: null));
}
}
/// An inherited widget that overrides the default icon of [BackButtonIcon],
/// [CloseButtonIcon], [DrawerButtonIcon], and [EndDrawerButtonIcon] in this
/// widget's subtree.
///
/// {@tool dartpad}
/// This example shows how to define custom builders for drawer and back
/// buttons.
///
/// ** See code in examples/api/lib/material/action_buttons/action_icon_theme.0.dart **
/// {@end-tool}
class ActionIconTheme extends InheritedTheme {
/// Creates a theme that overrides the default icon of [BackButtonIcon],
/// [CloseButtonIcon], [DrawerButtonIcon], and [EndDrawerButtonIcon] in this
/// widget's subtree.
const ActionIconTheme({
super.key,
required this.data,
required super.child,
});
/// Specifies the default icon overrides for descendant [BackButtonIcon],
/// [CloseButtonIcon], [DrawerButtonIcon], and [EndDrawerButtonIcon] widgets.
final ActionIconThemeData data;
/// The closest instance of this class that encloses the given context.
///
/// If there is no enclosing [ActionIconTheme] widget, then
/// [ThemeData.actionIconTheme] is used.
///
/// Typical usage is as follows:
///
/// ```dart
/// ActionIconThemeData? theme = ActionIconTheme.of(context);
/// ```
static ActionIconThemeData? of(BuildContext context) {
final ActionIconTheme? actionIconTheme = context.dependOnInheritedWidgetOfExactType<ActionIconTheme>();
return actionIconTheme?.data ?? Theme.of(context).actionIconTheme;
}
@override
Widget wrap(BuildContext context, Widget child) {
return ActionIconTheme(data: data, child: child);
}
@override
bool updateShouldNotify(ActionIconTheme oldWidget) => data != oldWidget.data;
}
| flutter/packages/flutter/lib/src/material/action_icons_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/action_icons_theme.dart",
"repo_id": "flutter",
"token_count": 1910
} | 647 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// AUTOGENERATED FILE DO NOT EDIT!
// This file was generated by vitool.
part of material_animated_icons; // ignore: use_string_in_part_of_directives
const _AnimatedIconData _$play_pause = _AnimatedIconData(
Size(48.0, 48.0),
<_PathFrames>[
_PathFrames(
opacities: <double>[
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(16.046875, 10.039062500000002),
Offset(16.316498427194905, 9.888877552610037),
Offset(17.350168694919763, 9.372654593279519),
Offset(19.411307079826894, 8.531523285503246),
Offset(22.581365240485308, 7.589125591600418),
Offset(25.499178877190392, 6.946027752843147),
Offset(28.464059662259196, 6.878006546805963),
Offset(30.817518246129985, 7.278084288616373),
Offset(32.55729037951853, 7.8522502852455425),
Offset(33.815177617779455, 8.44633949301522),
Offset(34.712260860180656, 8.99474841944718),
Offset(35.33082450786742, 9.453096000457315),
Offset(35.71938467416858, 9.764269500343072),
Offset(35.93041292728106, 9.940652668613495),
Offset(35.999770475547926, 9.999803268019111),
Offset(36.0, 10.0),
],
),
_PathCubicTo(
<Offset>[
Offset(16.046875, 10.039062500000002),
Offset(16.316498427194905, 9.888877552610037),
Offset(17.350168694919763, 9.372654593279519),
Offset(19.411307079826894, 8.531523285503246),
Offset(22.581365240485308, 7.589125591600418),
Offset(25.499178877190392, 6.946027752843147),
Offset(28.464059662259196, 6.878006546805963),
Offset(30.817518246129985, 7.278084288616373),
Offset(32.55729037951853, 7.8522502852455425),
Offset(33.815177617779455, 8.44633949301522),
Offset(34.712260860180656, 8.99474841944718),
Offset(35.33082450786742, 9.453096000457315),
Offset(35.71938467416858, 9.764269500343072),
Offset(35.93041292728106, 9.940652668613495),
Offset(35.999770475547926, 9.999803268019111),
Offset(36.0, 10.0),
],
<Offset>[
Offset(16.046875, 24.0),
Offset(16.048342217256838, 23.847239495401816),
Offset(16.077346902872737, 23.272630763824544),
Offset(16.048056811677085, 21.774352893256555),
Offset(16.312852147291277, 18.33792251536507),
Offset(17.783803270262858, 14.342870123090869),
Offset(20.317723014778526, 11.617364447163006),
Offset(22.6612333095366, 10.320666923510533),
Offset(24.489055761050455, 9.794101160418514),
Offset(25.820333134665205, 9.653975058221658),
Offset(26.739449095852216, 9.704987479092615),
Offset(27.339611564620206, 9.827950233030684),
Offset(27.720964836869285, 9.92326668993185),
Offset(27.930511332768496, 9.98033236260651),
Offset(27.999770476623045, 9.999934423927339),
Offset(27.999999999999996, 10.0),
],
<Offset>[
Offset(16.046875, 24.0),
Offset(16.048342217256838, 23.847239495401816),
Offset(16.077346902872737, 23.272630763824544),
Offset(16.048056811677085, 21.774352893256555),
Offset(16.312852147291277, 18.33792251536507),
Offset(17.783803270262858, 14.342870123090869),
Offset(20.317723014778526, 11.617364447163006),
Offset(22.6612333095366, 10.320666923510533),
Offset(24.489055761050455, 9.794101160418514),
Offset(25.820333134665205, 9.653975058221658),
Offset(26.739449095852216, 9.704987479092615),
Offset(27.339611564620206, 9.827950233030684),
Offset(27.720964836869285, 9.92326668993185),
Offset(27.930511332768496, 9.98033236260651),
Offset(27.999770476623045, 9.999934423927339),
Offset(27.999999999999996, 10.0),
],
),
_PathCubicTo(
<Offset>[
Offset(16.046875, 24.0),
Offset(16.048342217256838, 23.847239495401816),
Offset(16.077346902872737, 23.272630763824544),
Offset(16.048056811677085, 21.774352893256555),
Offset(16.312852147291277, 18.33792251536507),
Offset(17.783803270262858, 14.342870123090869),
Offset(20.317723014778526, 11.617364447163006),
Offset(22.6612333095366, 10.320666923510533),
Offset(24.489055761050455, 9.794101160418514),
Offset(25.820333134665205, 9.653975058221658),
Offset(26.739449095852216, 9.704987479092615),
Offset(27.339611564620206, 9.827950233030684),
Offset(27.720964836869285, 9.92326668993185),
Offset(27.930511332768496, 9.98033236260651),
Offset(27.999770476623045, 9.999934423927339),
Offset(27.999999999999996, 10.0),
],
<Offset>[
Offset(37.984375, 24.0),
Offset(37.98179511896882, 24.268606388242382),
Offset(37.92629019604922, 25.273340032354483),
Offset(37.60401862920776, 27.24886978355857),
Offset(36.59673961336577, 30.16713606026377),
Offset(35.26901818749416, 32.58105797429066),
Offset(33.66938906523204, 34.56713290494057),
Offset(32.196778918797094, 35.8827095523761),
Offset(30.969894470496282, 36.721466129987085),
Offset(29.989349224706995, 37.25388702486493),
Offset(29.223528593231507, 37.59010302049878),
Offset(28.651601378627003, 37.79719553439594),
Offset(28.27745500043001, 37.91773612047938),
Offset(28.069390261744058, 37.979987943400474),
Offset(28.000229522301836, 37.99993442016443),
Offset(28.0, 38.0),
],
<Offset>[
Offset(37.984375, 24.0),
Offset(37.98179511896882, 24.268606388242382),
Offset(37.92629019604922, 25.273340032354483),
Offset(37.60401862920776, 27.24886978355857),
Offset(36.59673961336577, 30.16713606026377),
Offset(35.26901818749416, 32.58105797429066),
Offset(33.66938906523204, 34.56713290494057),
Offset(32.196778918797094, 35.8827095523761),
Offset(30.969894470496282, 36.721466129987085),
Offset(29.989349224706995, 37.25388702486493),
Offset(29.223528593231507, 37.59010302049878),
Offset(28.651601378627003, 37.79719553439594),
Offset(28.27745500043001, 37.91773612047938),
Offset(28.069390261744058, 37.979987943400474),
Offset(28.000229522301836, 37.99993442016443),
Offset(28.0, 38.0),
],
),
_PathCubicTo(
<Offset>[
Offset(37.984375, 24.0),
Offset(37.98179511896882, 24.268606388242382),
Offset(37.92629019604922, 25.273340032354483),
Offset(37.60401862920776, 27.24886978355857),
Offset(36.59673961336577, 30.16713606026377),
Offset(35.26901818749416, 32.58105797429066),
Offset(33.66938906523204, 34.56713290494057),
Offset(32.196778918797094, 35.8827095523761),
Offset(30.969894470496282, 36.721466129987085),
Offset(29.989349224706995, 37.25388702486493),
Offset(29.223528593231507, 37.59010302049878),
Offset(28.651601378627003, 37.79719553439594),
Offset(28.27745500043001, 37.91773612047938),
Offset(28.069390261744058, 37.979987943400474),
Offset(28.000229522301836, 37.99993442016443),
Offset(28.0, 38.0),
],
<Offset>[
Offset(37.984375, 24.0),
Offset(37.98179511896882, 24.268606388242382),
Offset(37.92663369548548, 25.26958881281347),
Offset(37.702366207906195, 26.86162526614268),
Offset(37.62294586290445, 28.407471142252255),
Offset(38.43944238184115, 29.541526367903558),
Offset(38.93163276984633, 31.5056762828673),
Offset(38.80537374713073, 33.4174700441868),
Offset(38.35814295213548, 34.94327332096457),
Offset(37.78610517302408, 36.076173087300646),
Offset(37.186112675124534, 36.8807750697281),
Offset(36.64281432187422, 37.42234130182257),
Offset(36.275874837729305, 37.7587389308906),
Offset(36.06929185625662, 37.94030824940746),
Offset(36.00022952122672, 37.9998032642562),
Offset(36.0, 38.0),
],
<Offset>[
Offset(37.984375, 24.0),
Offset(37.98179511896882, 24.268606388242382),
Offset(37.92663369548548, 25.26958881281347),
Offset(37.702366207906195, 26.86162526614268),
Offset(37.62294586290445, 28.407471142252255),
Offset(38.43944238184115, 29.541526367903558),
Offset(38.93163276984633, 31.5056762828673),
Offset(38.80537374713073, 33.4174700441868),
Offset(38.35814295213548, 34.94327332096457),
Offset(37.78610517302408, 36.076173087300646),
Offset(37.186112675124534, 36.8807750697281),
Offset(36.64281432187422, 37.42234130182257),
Offset(36.275874837729305, 37.7587389308906),
Offset(36.06929185625662, 37.94030824940746),
Offset(36.00022952122672, 37.9998032642562),
Offset(36.0, 38.0),
],
),
_PathCubicTo(
<Offset>[
Offset(37.984375, 24.0),
Offset(37.98179511896882, 24.268606388242382),
Offset(37.92663369548548, 25.26958881281347),
Offset(37.702366207906195, 26.86162526614268),
Offset(37.62294586290445, 28.407471142252255),
Offset(38.43944238184115, 29.541526367903558),
Offset(38.93163276984633, 31.5056762828673),
Offset(38.80537374713073, 33.4174700441868),
Offset(38.35814295213548, 34.94327332096457),
Offset(37.78610517302408, 36.076173087300646),
Offset(37.186112675124534, 36.8807750697281),
Offset(36.64281432187422, 37.42234130182257),
Offset(36.275874837729305, 37.7587389308906),
Offset(36.06929185625662, 37.94030824940746),
Offset(36.00022952122672, 37.9998032642562),
Offset(36.0, 38.0),
],
<Offset>[
Offset(16.046875, 10.039062500000002),
Offset(16.316498427194905, 9.888877552610037),
Offset(17.35016869491465, 9.372654593335355),
Offset(19.411307079839695, 8.531523285452844),
Offset(22.58136524050546, 7.589125591565864),
Offset(25.499178877175954, 6.946027752856988),
Offset(28.464059662259196, 6.878006546805963),
Offset(30.817518246129985, 7.278084288616373),
Offset(32.55729037951755, 7.852250285245777),
Offset(33.81517761778539, 8.446339493014325),
Offset(34.71226086018563, 8.994748419446736),
Offset(35.33082450786742, 9.453096000457315),
Offset(35.71938467416858, 9.764269500343072),
Offset(35.93041292728106, 9.940652668613495),
Offset(35.999770475547926, 9.999803268019111),
Offset(36.0, 10.0),
],
<Offset>[
Offset(16.046875, 10.039062500000002),
Offset(16.316498427194905, 9.888877552610037),
Offset(17.35016869491465, 9.372654593335355),
Offset(19.411307079839695, 8.531523285452844),
Offset(22.58136524050546, 7.589125591565864),
Offset(25.499178877175954, 6.946027752856988),
Offset(28.464059662259196, 6.878006546805963),
Offset(30.817518246129985, 7.278084288616373),
Offset(32.55729037951755, 7.852250285245777),
Offset(33.81517761778539, 8.446339493014325),
Offset(34.71226086018563, 8.994748419446736),
Offset(35.33082450786742, 9.453096000457315),
Offset(35.71938467416858, 9.764269500343072),
Offset(35.93041292728106, 9.940652668613495),
Offset(35.999770475547926, 9.999803268019111),
Offset(36.0, 10.0),
],
),
_PathClose(
),
],
),
_PathFrames(
opacities: <double>[
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
1.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(37.984375, 24.0),
Offset(37.98179511896882, 24.268606388242382),
Offset(37.925946696573504, 25.277091251817644),
Offset(37.50567105053561, 27.636114300999704),
Offset(35.57053336387648, 31.926800978315658),
Offset(32.09859399311199, 35.6205895806324),
Offset(28.407145360613207, 37.6285895270458),
Offset(25.588184090469714, 38.34794906057932),
Offset(23.581645988882627, 38.49965893899394),
Offset(22.19259327642332, 38.43160096243417),
Offset(21.26094464377359, 38.29943245748053),
Offset(20.660388435379787, 38.17204976696931),
Offset(20.279035163130715, 38.07673331006816),
Offset(20.069488667231496, 38.01966763739349),
Offset(20.000229523376955, 38.00006557607266),
Offset(20.0, 38.0),
],
),
_PathCubicTo(
<Offset>[
Offset(37.984375, 24.0),
Offset(37.98179511896882, 24.268606388242382),
Offset(37.925946696573504, 25.277091251817644),
Offset(37.50567105053561, 27.636114300999704),
Offset(35.57053336387648, 31.926800978315658),
Offset(32.09859399311199, 35.6205895806324),
Offset(28.407145360613207, 37.6285895270458),
Offset(25.588184090469714, 38.34794906057932),
Offset(23.581645988882627, 38.49965893899394),
Offset(22.19259327642332, 38.43160096243417),
Offset(21.26094464377359, 38.29943245748053),
Offset(20.660388435379787, 38.17204976696931),
Offset(20.279035163130715, 38.07673331006816),
Offset(20.069488667231496, 38.01966763739349),
Offset(20.000229523376955, 38.00006557607266),
Offset(20.0, 38.0),
],
<Offset>[
Offset(16.046875, 24.0),
Offset(16.048342217256838, 23.847239495401816),
Offset(16.077003403397015, 23.276381983287706),
Offset(15.949709233004938, 22.161597410697688),
Offset(15.286645897801982, 20.097587433416958),
Offset(14.613379075880687, 17.38240172943261),
Offset(15.05547931015969, 14.678821069268237),
Offset(16.052638481209218, 12.785906431713748),
Offset(17.100807279436804, 11.57229396942536),
Offset(18.02357718638153, 10.831688995790898),
Offset(18.7768651463943, 10.414316916074366),
Offset(19.34839862137299, 10.202804465604057),
Offset(19.722544999569994, 10.082263879520628),
Offset(19.93060973825594, 10.02001205659953),
Offset(19.99977047769816, 10.000065579835564),
Offset(19.999999999999996, 10.000000000000004),
],
<Offset>[
Offset(16.046875, 24.0),
Offset(16.048342217256838, 23.847239495401816),
Offset(16.077003403397015, 23.276381983287706),
Offset(15.949709233004938, 22.161597410697688),
Offset(15.286645897801982, 20.097587433416958),
Offset(14.613379075880687, 17.38240172943261),
Offset(15.05547931015969, 14.678821069268237),
Offset(16.052638481209218, 12.785906431713748),
Offset(17.100807279436804, 11.57229396942536),
Offset(18.02357718638153, 10.831688995790898),
Offset(18.7768651463943, 10.414316916074366),
Offset(19.34839862137299, 10.202804465604057),
Offset(19.722544999569994, 10.082263879520628),
Offset(19.93060973825594, 10.02001205659953),
Offset(19.99977047769816, 10.000065579835564),
Offset(19.999999999999996, 10.000000000000004),
],
),
_PathCubicTo(
<Offset>[
Offset(16.046875, 24.0),
Offset(16.048342217256838, 23.847239495401816),
Offset(16.077003403397015, 23.276381983287706),
Offset(15.949709233004938, 22.161597410697688),
Offset(15.286645897801982, 20.097587433416958),
Offset(14.613379075880687, 17.38240172943261),
Offset(15.05547931015969, 14.678821069268237),
Offset(16.052638481209218, 12.785906431713748),
Offset(17.100807279436804, 11.57229396942536),
Offset(18.02357718638153, 10.831688995790898),
Offset(18.7768651463943, 10.414316916074366),
Offset(19.34839862137299, 10.202804465604057),
Offset(19.722544999569994, 10.082263879520628),
Offset(19.93060973825594, 10.02001205659953),
Offset(19.99977047769816, 10.000065579835564),
Offset(19.999999999999996, 10.000000000000004),
],
<Offset>[
Offset(16.046875, 37.9609375),
Offset(15.780186007318768, 37.8056014381936),
Offset(14.804181611349989, 37.17635815383272),
Offset(12.58645896485513, 35.404427018450995),
Offset(9.018132804607959, 30.846384357181606),
Offset(6.898003468953149, 24.77924409968033),
Offset(6.909142662679017, 19.41817896962528),
Offset(7.8963535446158275, 15.828489066607908),
Offset(9.032572660968736, 13.51414484459833),
Offset(10.02873270326728, 12.039324560997336),
Offset(10.80405338206586, 11.124555975719801),
Offset(11.357185678125777, 10.577658698177427),
Offset(11.724125162270699, 10.241261069109406),
Offset(11.930708143743377, 10.059691750592545),
Offset(11.999770478773279, 10.000196735743792),
Offset(11.999999999999996, 10.000000000000004),
],
<Offset>[
Offset(16.046875, 37.9609375),
Offset(15.780186007318768, 37.8056014381936),
Offset(14.804181611349989, 37.17635815383272),
Offset(12.58645896485513, 35.404427018450995),
Offset(9.018132804607959, 30.846384357181606),
Offset(6.898003468953149, 24.77924409968033),
Offset(6.909142662679017, 19.41817896962528),
Offset(7.8963535446158275, 15.828489066607908),
Offset(9.032572660968736, 13.51414484459833),
Offset(10.02873270326728, 12.039324560997336),
Offset(10.80405338206586, 11.124555975719801),
Offset(11.357185678125777, 10.577658698177427),
Offset(11.724125162270699, 10.241261069109406),
Offset(11.930708143743377, 10.059691750592545),
Offset(11.999770478773279, 10.000196735743792),
Offset(11.999999999999996, 10.000000000000004),
],
),
_PathCubicTo(
<Offset>[
Offset(16.046875, 37.9609375),
Offset(15.780186007318768, 37.8056014381936),
Offset(14.804181611349989, 37.17635815383272),
Offset(12.58645896485513, 35.404427018450995),
Offset(9.018132804607959, 30.846384357181606),
Offset(6.898003468953149, 24.77924409968033),
Offset(6.909142662679017, 19.41817896962528),
Offset(7.8963535446158275, 15.828489066607908),
Offset(9.032572660968736, 13.51414484459833),
Offset(10.02873270326728, 12.039324560997336),
Offset(10.80405338206586, 11.124555975719801),
Offset(11.357185678125777, 10.577658698177427),
Offset(11.724125162270699, 10.241261069109406),
Offset(11.930708143743377, 10.059691750592545),
Offset(11.999770478773279, 10.000196735743792),
Offset(11.999999999999996, 10.000000000000004),
],
<Offset>[
Offset(37.984375, 24.0),
Offset(37.98179511896882, 24.268606388242382),
Offset(37.92560319713213, 25.28084247141449),
Offset(37.40732347184997, 28.02335881836519),
Offset(34.544327114357955, 33.68646589629262),
Offset(28.928169798750567, 38.66012118703334),
Offset(23.144901655998915, 40.69004614911907),
Offset(18.979589262136074, 40.81318856876862),
Offset(16.193397507242462, 40.27785174801669),
Offset(14.395837328112165, 39.60931489999756),
Offset(13.298360561885538, 39.008760408250765),
Offset(12.669175492132574, 38.546903999542685),
Offset(12.280615325831423, 38.23573049965694),
Offset(12.069587072718935, 38.05934733138651),
Offset(12.000229524452074, 38.00019673198088),
Offset(12.0, 38.0),
],
<Offset>[
Offset(37.984375, 24.0),
Offset(37.98179511896882, 24.268606388242382),
Offset(37.92560319713213, 25.28084247141449),
Offset(37.40732347184997, 28.02335881836519),
Offset(34.544327114357955, 33.68646589629262),
Offset(28.928169798750567, 38.66012118703334),
Offset(23.144901655998915, 40.69004614911907),
Offset(18.979589262136074, 40.81318856876862),
Offset(16.193397507242462, 40.27785174801669),
Offset(14.395837328112165, 39.60931489999756),
Offset(13.298360561885538, 39.008760408250765),
Offset(12.669175492132574, 38.546903999542685),
Offset(12.280615325831423, 38.23573049965694),
Offset(12.069587072718935, 38.05934733138651),
Offset(12.000229524452074, 38.00019673198088),
Offset(12.0, 38.0),
],
),
_PathCubicTo(
<Offset>[
Offset(37.984375, 24.0),
Offset(37.98179511896882, 24.268606388242382),
Offset(37.92560319713213, 25.28084247141449),
Offset(37.40732347184997, 28.02335881836519),
Offset(34.544327114357955, 33.68646589629262),
Offset(28.928169798750567, 38.66012118703334),
Offset(23.144901655998915, 40.69004614911907),
Offset(18.979589262136074, 40.81318856876862),
Offset(16.193397507242462, 40.27785174801669),
Offset(14.395837328112165, 39.60931489999756),
Offset(13.298360561885538, 39.008760408250765),
Offset(12.669175492132574, 38.546903999542685),
Offset(12.280615325831423, 38.23573049965694),
Offset(12.069587072718935, 38.05934733138651),
Offset(12.000229524452074, 38.00019673198088),
Offset(12.0, 38.0),
],
<Offset>[
Offset(37.984375, 24.0),
Offset(37.98179511896882, 24.268606388242382),
Offset(37.92594669656839, 25.27709125187348),
Offset(37.50567105054841, 27.636114300949302),
Offset(35.57053336389663, 31.9268009782811),
Offset(32.09859399309755, 35.62058958064624),
Offset(28.407145360613207, 37.628589527045804),
Offset(25.588184090469714, 38.34794906057932),
Offset(23.58164598888166, 38.49965893899417),
Offset(22.192593276429257, 38.43160096243327),
Offset(21.260944643778565, 38.29943245748009),
Offset(20.660388435379787, 38.17204976696931),
Offset(20.279035163130715, 38.07673331006816),
Offset(20.069488667231496, 38.01966763739349),
Offset(20.000229523376955, 38.00006557607266),
Offset(20.0, 38.0),
],
<Offset>[
Offset(37.984375, 24.0),
Offset(37.98179511896882, 24.268606388242382),
Offset(37.92594669656839, 25.27709125187348),
Offset(37.50567105054841, 27.636114300949302),
Offset(35.57053336389663, 31.9268009782811),
Offset(32.09859399309755, 35.62058958064624),
Offset(28.407145360613207, 37.628589527045804),
Offset(25.588184090469714, 38.34794906057932),
Offset(23.58164598888166, 38.49965893899417),
Offset(22.192593276429257, 38.43160096243327),
Offset(21.260944643778565, 38.29943245748009),
Offset(20.660388435379787, 38.17204976696931),
Offset(20.279035163130715, 38.07673331006816),
Offset(20.069488667231496, 38.01966763739349),
Offset(20.000229523376955, 38.00006557607266),
Offset(20.0, 38.0),
],
),
_PathClose(
),
],
),
_PathFrames(
opacities: <double>[
1.0,
1.0,
1.0,
1.0,
0.733333333333,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
0.0,
],
commands: <_PathCommand>[
_PathMoveTo(
<Offset>[
Offset(36.21875, 24.387283325200002),
Offset(36.858953419818775, 24.63439009154731),
Offset(37.42714268809582, 25.618428032998864),
Offset(37.46673246436919, 27.957602694496682),
Offset(35.51445214909996, 31.937043103050268),
Offset(32.888668544302234, 34.79679735028506),
Offset(30.100083850883422, 36.58444430738925),
Offset(27.884884986535624, 37.434542424473584),
Offset(26.23678799810123, 37.80492814052796),
Offset(25.03902259291319, 37.946314694750235),
Offset(24.185908910024594, 37.98372980970255),
Offset(23.59896217337824, 37.97921421880389),
Offset(23.221743554700737, 37.96329396736102),
Offset(23.013561704380457, 37.95013265178958),
Offset(22.94461033630511, 37.9450856638228),
Offset(22.9443817139, 37.945068359375),
],
),
_PathCubicTo(
<Offset>[
Offset(36.21875, 24.387283325200002),
Offset(36.858953419818775, 24.63439009154731),
Offset(37.42714268809582, 25.618428032998864),
Offset(37.46673246436919, 27.957602694496682),
Offset(35.51445214909996, 31.937043103050268),
Offset(32.888668544302234, 34.79679735028506),
Offset(30.100083850883422, 36.58444430738925),
Offset(27.884884986535624, 37.434542424473584),
Offset(26.23678799810123, 37.80492814052796),
Offset(25.03902259291319, 37.946314694750235),
Offset(24.185908910024594, 37.98372980970255),
Offset(23.59896217337824, 37.97921421880389),
Offset(23.221743554700737, 37.96329396736102),
Offset(23.013561704380457, 37.95013265178958),
Offset(22.94461033630511, 37.9450856638228),
Offset(22.9443817139, 37.945068359375),
],
<Offset>[
Offset(36.1819000244141, 23.597152709966),
Offset(36.8358384608093, 23.843669618675563),
Offset(37.45961204802207, 24.827964901265894),
Offset(37.71106940406011, 26.916549745564488),
Offset(36.67279396166709, 30.08280087402087),
Offset(34.51215067847019, 33.33246277147643),
Offset(32.022419367141104, 35.54300484126963),
Offset(29.955608739426065, 36.73306317469314),
Offset(28.376981306736234, 37.3582262261251),
Offset(27.209745307333925, 37.68567529681684),
Offset(26.368492376458054, 37.856060664218916),
Offset(25.784980483216092, 37.94324273411291),
Offset(25.407936267815487, 37.98634651128109),
Offset(25.199167384595825, 38.0057906185826),
Offset(25.129914160588893, 38.01154763962766),
Offset(25.129684448280003, 38.0115661621094),
],
<Offset>[
Offset(36.1819000244141, 23.597152709966),
Offset(36.8358384608093, 23.843669618675563),
Offset(37.45961204802207, 24.827964901265894),
Offset(37.71106940406011, 26.916549745564488),
Offset(36.67279396166709, 30.08280087402087),
Offset(34.51215067847019, 33.33246277147643),
Offset(32.022419367141104, 35.54300484126963),
Offset(29.955608739426065, 36.73306317469314),
Offset(28.376981306736234, 37.3582262261251),
Offset(27.209745307333925, 37.68567529681684),
Offset(26.368492376458054, 37.856060664218916),
Offset(25.784980483216092, 37.94324273411291),
Offset(25.407936267815487, 37.98634651128109),
Offset(25.199167384595825, 38.0057906185826),
Offset(25.129914160588893, 38.01154763962766),
Offset(25.129684448280003, 38.0115661621094),
],
),
_PathCubicTo(
<Offset>[
Offset(36.1819000244141, 23.597152709966),
Offset(36.8358384608093, 23.843669618675563),
Offset(37.45961204802207, 24.827964901265894),
Offset(37.71106940406011, 26.916549745564488),
Offset(36.67279396166709, 30.08280087402087),
Offset(34.51215067847019, 33.33246277147643),
Offset(32.022419367141104, 35.54300484126963),
Offset(29.955608739426065, 36.73306317469314),
Offset(28.376981306736234, 37.3582262261251),
Offset(27.209745307333925, 37.68567529681684),
Offset(26.368492376458054, 37.856060664218916),
Offset(25.784980483216092, 37.94324273411291),
Offset(25.407936267815487, 37.98634651128109),
Offset(25.199167384595825, 38.0057906185826),
Offset(25.129914160588893, 38.01154763962766),
Offset(25.129684448280003, 38.0115661621094),
],
<Offset>[
Offset(16.1149902344141, 22.955383300786004),
Offset(15.997629933953313, 22.801455805116497),
Offset(15.966446205406928, 22.215379763234004),
Offset(16.088459709151728, 20.876736411055298),
Offset(16.769441289779344, 18.37084947089115),
Offset(18.595653610551377, 16.59990844352802),
Offset(20.48764499639903, 15.536450078720307),
Offset(21.968961727208672, 15.064497861016925),
Offset(23.06110116092593, 14.884804779309462),
Offset(23.849967628988242, 14.837805654268031),
Offset(24.40943781230773, 14.84572910499329),
Offset(24.793207208324446, 14.870972819299066),
Offset(25.03935354219434, 14.895712045654406),
Offset(25.1750322217718, 14.912227213496571),
Offset(25.21994388130627, 14.918147112632923),
Offset(25.220092773475297, 14.9181671142094),
],
<Offset>[
Offset(16.170043945314102, 22.942321777349),
Offset(16.055083258838646, 22.789495616149246),
Offset(16.026762188208856, 22.207786731939372),
Offset(16.150920741832245, 20.879123319500057),
Offset(16.82882476693832, 18.390360508490243),
Offset(18.647384744725734, 16.634993592875272),
Offset(20.52967353640347, 15.58271755944683),
Offset(22.002563841255288, 15.117204368008782),
Offset(23.0881035089048, 14.941178098808251),
Offset(23.872012376061566, 14.896295884855345),
Offset(24.42787166552447, 14.90545574061985),
Offset(24.80911858591767, 14.931420366898372),
Offset(25.053627357583, 14.956567087696417),
Offset(25.188396770682292, 14.973288385939487),
Offset(25.233006406883348, 14.979273607487709),
Offset(25.233154296913, 14.9792938232094),
],
),
_PathCubicTo(
<Offset>[
Offset(16.225097656251602, 22.9292602539115),
Offset(16.112536583755883, 22.7775354271821),
Offset(16.087078170937534, 22.200193700637527),
Offset(16.213381774594694, 20.88151022796511),
Offset(16.888208244083728, 18.409871546081646),
Offset(18.699115878889145, 16.67007874221141),
Offset(20.571702076399895, 15.628985040159975),
Offset(22.03616595529626, 15.16991087498609),
Offset(23.115105856879826, 14.997551418291916),
Offset(23.894057123132363, 14.954786115427265),
Offset(24.446305518739628, 14.965182376230889),
Offset(24.825029963509966, 14.9918679144821),
Offset(25.067901172971148, 15.017422129722831),
Offset(25.201761319592507, 15.034349558366799),
Offset(25.24606893246022, 15.040400102326899),
Offset(25.2462158203505, 15.0404205321938),
],
<Offset>[
Offset(16.172653198243793, 25.050704956059),
Offset(16.017298096111325, 24.897541931224776),
Offset(15.837305455486472, 24.307642370134865),
Offset(15.617771431142284, 23.034739327639596),
Offset(15.534079923477577, 20.72510957725349),
Offset(16.76065281331448, 18.52381863579275),
Offset(18.25163791556585, 16.97482787617967),
Offset(19.521978435885586, 16.104176237124552),
Offset(20.506617505527394, 15.621874388004521),
Offset(21.24147683283453, 15.352037236477383),
Offset(21.774425023577333, 15.199799658679147),
Offset(22.14565785051594, 15.114161535583197),
Offset(22.386204205776483, 15.067342323943635),
Offset(22.519618086537456, 15.044265557010121),
Offset(22.563909453457644, 15.037056623787358),
Offset(22.564056396523, 15.0370330810219),
],
<Offset>[
Offset(16.172653198243804, 25.050704956059),
Offset(16.017298096111343, 24.89754193122478),
Offset(15.837305455486483, 24.307642370134865),
Offset(15.617771431142284, 23.034739327639596),
Offset(15.534079923477577, 20.72510957725349),
Offset(16.76065281331448, 18.52381863579275),
Offset(18.25163791556585, 16.97482787617967),
Offset(19.521978435885586, 16.104176237124552),
Offset(20.506617505527394, 15.621874388004521),
Offset(21.24147683283453, 15.352037236477383),
Offset(21.774425023577333, 15.199799658679147),
Offset(22.14565785051594, 15.114161535583197),
Offset(22.386204205776483, 15.067342323943635),
Offset(22.519618086537456, 15.044265557010121),
Offset(22.563909453457644, 15.037056623787358),
Offset(22.564056396523, 15.0370330810219),
],
),
_PathCubicTo(
<Offset>[
Offset(16.172653198243804, 25.050704956059),
Offset(16.017298096111343, 24.89754193122478),
Offset(15.837305455486483, 24.307642370134865),
Offset(15.617771431142284, 23.034739327639596),
Offset(15.534079923477577, 20.72510957725349),
Offset(16.76065281331448, 18.52381863579275),
Offset(18.25163791556585, 16.97482787617967),
Offset(19.521978435885586, 16.104176237124552),
Offset(20.506617505527394, 15.621874388004521),
Offset(21.24147683283453, 15.352037236477383),
Offset(21.774425023577333, 15.199799658679147),
Offset(22.14565785051594, 15.114161535583197),
Offset(22.386204205776483, 15.067342323943635),
Offset(22.519618086537456, 15.044265557010121),
Offset(22.563909453457644, 15.037056623787358),
Offset(22.564056396523, 15.0370330810219),
],
<Offset>[
Offset(36.218750000043805, 24.387283325200002),
Offset(36.858953419751415, 24.634390091546017),
Offset(37.42714268811728, 25.61842803300083),
Offset(37.46673246430412, 27.95760269448635),
Offset(35.51445214905712, 31.937043103018333),
Offset(32.88866854426982, 34.79679735024258),
Offset(30.100083850861907, 36.584444307340334),
Offset(27.884884986522685, 37.434542424421736),
Offset(26.23678799809464, 37.80492814047493),
Offset(25.039022592911195, 37.94631469469684),
Offset(24.185908910025862, 37.983729809649134),
Offset(23.59896217338175, 37.97921421875057),
Offset(23.221743554705682, 37.96329396730781),
Offset(23.0135617043862, 37.95013265173645),
Offset(22.94461033631111, 37.9450856637697),
Offset(22.944381713906004, 37.9450683593219),
],
<Offset>[
Offset(36.218750000043805, 24.387283325200002),
Offset(36.858953419751415, 24.634390091546017),
Offset(37.42714268811728, 25.61842803300083),
Offset(37.46673246430412, 27.95760269448635),
Offset(35.51445214905712, 31.937043103018333),
Offset(32.88866854426982, 34.79679735024258),
Offset(30.100083850861907, 36.584444307340334),
Offset(27.884884986522685, 37.434542424421736),
Offset(26.23678799809464, 37.80492814047493),
Offset(25.039022592911195, 37.94631469469684),
Offset(24.185908910025862, 37.983729809649134),
Offset(23.59896217338175, 37.97921421875057),
Offset(23.221743554705682, 37.96329396730781),
Offset(23.0135617043862, 37.95013265173645),
Offset(22.94461033631111, 37.9450856637697),
Offset(22.944381713906004, 37.9450683593219),
],
),
_PathClose(
),
],
),
],
);
| flutter/packages/flutter/lib/src/material/animated_icons/data/play_pause.g.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/animated_icons/data/play_pause.g.dart",
"repo_id": "flutter",
"token_count": 22763
} | 648 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'bottom_navigation_bar.dart';
import 'material_state.dart';
import 'theme.dart';
// Examples can assume:
// late BuildContext context;
/// Defines default property values for descendant [BottomNavigationBar]
/// widgets.
///
/// Descendant widgets obtain the current [BottomNavigationBarThemeData] object
/// using `BottomNavigationBarTheme.of(context)`. Instances of
/// [BottomNavigationBarThemeData] can be customized with
/// [BottomNavigationBarThemeData.copyWith].
///
/// Typically a [BottomNavigationBarThemeData] is specified as part of the
/// overall [Theme] with [ThemeData.bottomNavigationBarTheme].
///
/// All [BottomNavigationBarThemeData] properties are `null` by default. When
/// null, the [BottomNavigationBar]'s build method provides defaults.
///
/// See also:
///
/// * [ThemeData], which describes the overall theme information for the
/// application.
@immutable
class BottomNavigationBarThemeData with Diagnosticable {
/// Creates a theme that can be used for [ThemeData.bottomNavigationBarTheme].
const BottomNavigationBarThemeData({
this.backgroundColor,
this.elevation,
this.selectedIconTheme,
this.unselectedIconTheme,
this.selectedItemColor,
this.unselectedItemColor,
this.selectedLabelStyle,
this.unselectedLabelStyle,
this.showSelectedLabels,
this.showUnselectedLabels,
this.type,
this.enableFeedback,
this.landscapeLayout,
this.mouseCursor,
});
/// The color of the [BottomNavigationBar] itself.
///
/// See [BottomNavigationBar.backgroundColor].
final Color? backgroundColor;
/// The z-coordinate of the [BottomNavigationBar].
///
/// See [BottomNavigationBar.elevation].
final double? elevation;
/// The size, opacity, and color of the icon in the currently selected
/// [BottomNavigationBarItem.icon].
///
/// If [BottomNavigationBar.selectedIconTheme] is non-null on the widget,
/// the whole [IconThemeData] from the widget will be used over this
/// [selectedIconTheme].
///
/// See [BottomNavigationBar.selectedIconTheme].
final IconThemeData? selectedIconTheme;
/// The size, opacity, and color of the icon in the currently unselected
/// [BottomNavigationBarItem.icon]s.
///
/// If [BottomNavigationBar.unselectedIconTheme] is non-null on the widget,
/// the whole [IconThemeData] from the widget will be used over this
/// [unselectedIconTheme].
///
/// See [BottomNavigationBar.unselectedIconTheme].
final IconThemeData? unselectedIconTheme;
/// The color of the selected [BottomNavigationBarItem.icon] and
/// [BottomNavigationBarItem.label].
///
/// See [BottomNavigationBar.selectedItemColor].
final Color? selectedItemColor;
/// The color of the unselected [BottomNavigationBarItem.icon] and
/// [BottomNavigationBarItem.label]s.
///
/// See [BottomNavigationBar.unselectedItemColor].
final Color? unselectedItemColor;
/// The [TextStyle] of the [BottomNavigationBarItem] labels when they are
/// selected.
///
/// See [BottomNavigationBar.selectedLabelStyle].
final TextStyle? selectedLabelStyle;
/// The [TextStyle] of the [BottomNavigationBarItem] labels when they are not
/// selected.
///
/// See [BottomNavigationBar.unselectedLabelStyle].
final TextStyle? unselectedLabelStyle;
/// Whether the labels are shown for the selected [BottomNavigationBarItem].
///
/// See [BottomNavigationBar.showSelectedLabels].
final bool? showSelectedLabels;
/// Whether the labels are shown for the unselected [BottomNavigationBarItem]s.
///
/// See [BottomNavigationBar.showUnselectedLabels].
final bool? showUnselectedLabels;
/// Defines the layout and behavior of a [BottomNavigationBar].
///
/// See [BottomNavigationBar.type].
final BottomNavigationBarType? type;
/// If specified, defines the feedback property for [BottomNavigationBar].
///
/// If [BottomNavigationBar.enableFeedback] is provided, [enableFeedback] is ignored.
final bool? enableFeedback;
/// If non-null, overrides the [BottomNavigationBar.landscapeLayout] property.
final BottomNavigationBarLandscapeLayout? landscapeLayout;
/// If specified, overrides the default value of [BottomNavigationBar.mouseCursor].
final MaterialStateProperty<MouseCursor?>? mouseCursor;
/// Creates a copy of this object but with the given fields replaced with the
/// new values.
BottomNavigationBarThemeData copyWith({
Color? backgroundColor,
double? elevation,
IconThemeData? selectedIconTheme,
IconThemeData? unselectedIconTheme,
Color? selectedItemColor,
Color? unselectedItemColor,
TextStyle? selectedLabelStyle,
TextStyle? unselectedLabelStyle,
bool? showSelectedLabels,
bool? showUnselectedLabels,
BottomNavigationBarType? type,
bool? enableFeedback,
BottomNavigationBarLandscapeLayout? landscapeLayout,
MaterialStateProperty<MouseCursor?>? mouseCursor,
}) {
return BottomNavigationBarThemeData(
backgroundColor: backgroundColor ?? this.backgroundColor,
elevation: elevation ?? this.elevation,
selectedIconTheme: selectedIconTheme ?? this.selectedIconTheme,
unselectedIconTheme: unselectedIconTheme ?? this.unselectedIconTheme,
selectedItemColor: selectedItemColor ?? this.selectedItemColor,
unselectedItemColor: unselectedItemColor ?? this.unselectedItemColor,
selectedLabelStyle: selectedLabelStyle ?? this.selectedLabelStyle,
unselectedLabelStyle: unselectedLabelStyle ?? this.unselectedLabelStyle,
showSelectedLabels: showSelectedLabels ?? this.showSelectedLabels,
showUnselectedLabels: showUnselectedLabels ?? this.showUnselectedLabels,
type: type ?? this.type,
enableFeedback: enableFeedback ?? this.enableFeedback,
landscapeLayout: landscapeLayout ?? this.landscapeLayout,
mouseCursor: mouseCursor ?? this.mouseCursor,
);
}
/// Linearly interpolate between two [BottomNavigationBarThemeData].
///
/// {@macro dart.ui.shadow.lerp}
static BottomNavigationBarThemeData lerp(BottomNavigationBarThemeData? a, BottomNavigationBarThemeData? b, double t) {
if (identical(a, b) && a != null) {
return a;
}
return BottomNavigationBarThemeData(
backgroundColor: Color.lerp(a?.backgroundColor, b?.backgroundColor, t),
elevation: lerpDouble(a?.elevation, b?.elevation, t),
selectedIconTheme: IconThemeData.lerp(a?.selectedIconTheme, b?.selectedIconTheme, t),
unselectedIconTheme: IconThemeData.lerp(a?.unselectedIconTheme, b?.unselectedIconTheme, t),
selectedItemColor: Color.lerp(a?.selectedItemColor, b?.selectedItemColor, t),
unselectedItemColor: Color.lerp(a?.unselectedItemColor, b?.unselectedItemColor, t),
selectedLabelStyle: TextStyle.lerp(a?.selectedLabelStyle, b?.selectedLabelStyle, t),
unselectedLabelStyle: TextStyle.lerp(a?.unselectedLabelStyle, b?.unselectedLabelStyle, t),
showSelectedLabels: t < 0.5 ? a?.showSelectedLabels : b?.showSelectedLabels,
showUnselectedLabels: t < 0.5 ? a?.showUnselectedLabels : b?.showUnselectedLabels,
type: t < 0.5 ? a?.type : b?.type,
enableFeedback: t < 0.5 ? a?.enableFeedback : b?.enableFeedback,
landscapeLayout: t < 0.5 ? a?.landscapeLayout : b?.landscapeLayout,
mouseCursor: t < 0.5 ? a?.mouseCursor : b?.mouseCursor,
);
}
@override
int get hashCode => Object.hash(
backgroundColor,
elevation,
selectedIconTheme,
unselectedIconTheme,
selectedItemColor,
unselectedItemColor,
selectedLabelStyle,
unselectedLabelStyle,
showSelectedLabels,
showUnselectedLabels,
type,
enableFeedback,
landscapeLayout,
mouseCursor,
);
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is BottomNavigationBarThemeData
&& other.backgroundColor == backgroundColor
&& other.elevation == elevation
&& other.selectedIconTheme == selectedIconTheme
&& other.unselectedIconTheme == unselectedIconTheme
&& other.selectedItemColor == selectedItemColor
&& other.unselectedItemColor == unselectedItemColor
&& other.selectedLabelStyle == selectedLabelStyle
&& other.unselectedLabelStyle == unselectedLabelStyle
&& other.showSelectedLabels == showSelectedLabels
&& other.showUnselectedLabels == showUnselectedLabels
&& other.type == type
&& other.enableFeedback == enableFeedback
&& other.landscapeLayout == landscapeLayout
&& other.mouseCursor == mouseCursor;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(ColorProperty('backgroundColor', backgroundColor, defaultValue: null));
properties.add(DoubleProperty('elevation', elevation, defaultValue: null));
properties.add(DiagnosticsProperty<IconThemeData>('selectedIconTheme', selectedIconTheme, defaultValue: null));
properties.add(DiagnosticsProperty<IconThemeData>('unselectedIconTheme', unselectedIconTheme, defaultValue: null));
properties.add(ColorProperty('selectedItemColor', selectedItemColor, defaultValue: null));
properties.add(ColorProperty('unselectedItemColor', unselectedItemColor, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('selectedLabelStyle', selectedLabelStyle, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('unselectedLabelStyle', unselectedLabelStyle, defaultValue: null));
properties.add(DiagnosticsProperty<bool>('showSelectedLabels', showSelectedLabels, defaultValue: null));
properties.add(DiagnosticsProperty<bool>('showUnselectedLabels', showUnselectedLabels, defaultValue: null));
properties.add(DiagnosticsProperty<BottomNavigationBarType>('type', type, defaultValue: null));
properties.add(DiagnosticsProperty<bool>('enableFeedback', enableFeedback, defaultValue: null));
properties.add(DiagnosticsProperty<BottomNavigationBarLandscapeLayout>('landscapeLayout', landscapeLayout, defaultValue: null));
properties.add(DiagnosticsProperty<MaterialStateProperty<MouseCursor?>>('mouseCursor', mouseCursor, defaultValue: null));
}
}
/// Applies a bottom navigation bar theme to descendant [BottomNavigationBar]
/// widgets.
///
/// Descendant widgets obtain the current theme's [BottomNavigationBarTheme]
/// object using [BottomNavigationBarTheme.of]. When a widget uses
/// [BottomNavigationBarTheme.of], it is automatically rebuilt if the theme
/// later changes.
///
/// A bottom navigation theme can be specified as part of the overall Material
/// theme using [ThemeData.bottomNavigationBarTheme].
///
/// See also:
///
/// * [BottomNavigationBarThemeData], which describes the actual configuration
/// of a bottom navigation bar theme.
class BottomNavigationBarTheme extends InheritedWidget {
/// Constructs a bottom navigation bar theme that configures all descendant
/// [BottomNavigationBar] widgets.
const BottomNavigationBarTheme({
super.key,
required this.data,
required super.child,
});
/// The properties used for all descendant [BottomNavigationBar] widgets.
final BottomNavigationBarThemeData data;
/// Returns the configuration [data] from the closest
/// [BottomNavigationBarTheme] ancestor. If there is no ancestor, it returns
/// [ThemeData.bottomNavigationBarTheme]. Applications can assume that the
/// returned value will not be null.
///
/// Typical usage is as follows:
///
/// ```dart
/// BottomNavigationBarThemeData theme = BottomNavigationBarTheme.of(context);
/// ```
static BottomNavigationBarThemeData of(BuildContext context) {
final BottomNavigationBarTheme? bottomNavTheme = context.dependOnInheritedWidgetOfExactType<BottomNavigationBarTheme>();
return bottomNavTheme?.data ?? Theme.of(context).bottomNavigationBarTheme;
}
@override
bool updateShouldNotify(BottomNavigationBarTheme oldWidget) => data != oldWidget.data;
}
| flutter/packages/flutter/lib/src/material/bottom_navigation_bar_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/bottom_navigation_bar_theme.dart",
"repo_id": "flutter",
"token_count": 3800
} | 649 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'colors.dart';
import 'material_state.dart';
import 'theme.dart';
/// Applies a chip theme to descendant [RawChip]-based widgets, like [Chip],
/// [InputChip], [ChoiceChip], [FilterChip], and [ActionChip].
///
/// A chip theme describes the color, shape and text styles for the chips it is
/// applied to.
///
/// Descendant widgets obtain the current theme's [ChipThemeData] object using
/// [ChipTheme.of]. When a widget uses [ChipTheme.of], it is automatically
/// rebuilt if the theme later changes.
///
/// The [ThemeData] object given by the [Theme.of] call also contains a default
/// [ThemeData.chipTheme] that can be customized by copying it (using
/// [ChipThemeData.copyWith]).
///
/// See also:
///
/// * [Chip], a chip that displays information and can be deleted.
/// * [InputChip], a chip that represents a complex piece of information, such
/// as an entity (person, place, or thing) or conversational text, in a
/// compact form.
/// * [ChoiceChip], allows a single selection from a set of options. Choice
/// chips contain related descriptive text or categories.
/// * [FilterChip], uses tags or descriptive words as a way to filter content.
/// * [ActionChip], represents an action related to primary content.
/// * [ChipThemeData], which describes the actual configuration of a chip
/// theme.
/// * [ThemeData], which describes the overall theme information for the
/// application.
class ChipTheme extends InheritedTheme {
/// Applies the given theme [data] to [child].
const ChipTheme({
super.key,
required this.data,
required super.child,
});
/// Specifies the color, shape, and text style values for descendant chip
/// widgets.
final ChipThemeData data;
/// Returns the data from the closest [ChipTheme] instance that encloses
/// the given context.
///
/// Defaults to the ambient [ThemeData.chipTheme] if there is no
/// [ChipTheme] in the given build context.
///
/// {@tool snippet}
///
/// ```dart
/// class Spaceship extends StatelessWidget {
/// const Spaceship({super.key});
///
/// @override
/// Widget build(BuildContext context) {
/// return ChipTheme(
/// data: ChipTheme.of(context).copyWith(backgroundColor: Colors.red),
/// child: ActionChip(
/// label: const Text('Launch'),
/// onPressed: () { print('We have liftoff!'); },
/// ),
/// );
/// }
/// }
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [ChipThemeData], which describes the actual configuration of a chip
/// theme.
static ChipThemeData of(BuildContext context) {
final ChipTheme? inheritedTheme = context.dependOnInheritedWidgetOfExactType<ChipTheme>();
return inheritedTheme?.data ?? Theme.of(context).chipTheme;
}
@override
Widget wrap(BuildContext context, Widget child) {
return ChipTheme(data: data, child: child);
}
@override
bool updateShouldNotify(ChipTheme oldWidget) => data != oldWidget.data;
}
/// Holds the color, shape, and text styles for a Material Design chip theme.
///
/// Use this class to configure a [ChipTheme] widget, or to set the
/// [ThemeData.chipTheme] for a [Theme] widget.
///
/// To obtain the current ambient chip theme, use [ChipTheme.of].
///
/// The parts of a chip are:
///
/// * The "avatar", which is a widget that appears at the beginning of the
/// chip. This is typically a [CircleAvatar] widget.
/// * The "label", which is the widget displayed in the center of the chip.
/// Typically this is a [Text] widget.
/// * The "delete icon", which is a widget that appears at the end of the chip.
/// * The chip is disabled when it is not accepting user input. Only some chips
/// have a disabled state: [ActionChip], [ChoiceChip], [FilterChip], and
/// [InputChip].
///
/// The simplest way to create a ChipThemeData is to use [copyWith] on the one
/// you get from [ChipTheme.of], or create an entirely new one with
/// [ChipThemeData.fromDefaults].
///
/// {@tool snippet}
///
/// ```dart
/// class CarColor extends StatefulWidget {
/// const CarColor({super.key});
///
/// @override
/// State createState() => _CarColorState();
/// }
///
/// class _CarColorState extends State<CarColor> {
/// Color _color = Colors.red;
///
/// @override
/// Widget build(BuildContext context) {
/// return ChipTheme(
/// data: ChipTheme.of(context).copyWith(backgroundColor: Colors.lightBlue),
/// child: ChoiceChip(
/// label: const Text('Light Blue'),
/// onSelected: (bool value) {
/// setState(() {
/// _color = value ? Colors.lightBlue : Colors.red;
/// });
/// },
/// selected: _color == Colors.lightBlue,
/// ),
/// );
/// }
/// }
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [Chip], a chip that displays information and can be deleted.
/// * [InputChip], a chip that represents a complex piece of information, such
/// as an entity (person, place, or thing) or conversational text, in a
/// compact form.
/// * [ChoiceChip], allows a single selection from a set of options. Choice
/// chips contain related descriptive text or categories.
/// * [FilterChip], uses tags or descriptive words as a way to filter content.
/// * [ActionChip], represents an action related to primary content.
/// * [CircleAvatar], which shows images or initials of entities.
/// * [Wrap], A widget that displays its children in multiple horizontal or
/// vertical runs.
/// * [ChipTheme] widget, which can override the chip theme of its
/// children.
/// * [Theme] widget, which performs a similar function to [ChipTheme],
/// but for overall themes.
/// * [ThemeData], which has a default [ChipThemeData].
@immutable
class ChipThemeData with Diagnosticable {
/// Create a [ChipThemeData] given a set of exact values. All the values
/// must be specified except for [shadowColor], [selectedShadowColor],
/// [elevation], and [pressElevation], which may be null.
///
/// This will rarely be used directly. It is used by [lerp] to
/// create intermediate themes based on two themes.
const ChipThemeData({
this.color,
this.backgroundColor,
this.deleteIconColor,
this.disabledColor,
this.selectedColor,
this.secondarySelectedColor,
this.shadowColor,
this.surfaceTintColor,
this.selectedShadowColor,
this.showCheckmark,
this.checkmarkColor,
this.labelPadding,
this.padding,
this.side,
this.shape,
this.labelStyle,
this.secondaryLabelStyle,
this.brightness,
this.elevation,
this.pressElevation,
this.iconTheme,
this.avatarBoxConstraints,
this.deleteIconBoxConstraints,
});
/// Generates a ChipThemeData from a brightness, a primary color, and a text
/// style.
///
/// The [brightness] is used to select a primary color from the default
/// values.
///
/// The optional [primaryColor] is used as the base color for the other
/// colors. The opacity of the [primaryColor] is ignored. If a [primaryColor]
/// is specified, then the [brightness] is ignored, and the theme brightness
/// is determined from the [primaryColor].
///
/// Only one of [primaryColor] or [brightness] may be specified.
///
/// The [secondaryColor] is used for the selection colors needed by
/// [ChoiceChip].
///
/// This is used to generate the default chip theme for a [ThemeData].
factory ChipThemeData.fromDefaults({
Brightness? brightness,
Color? primaryColor,
required Color secondaryColor,
required TextStyle labelStyle,
}) {
assert(primaryColor != null || brightness != null, 'One of primaryColor or brightness must be specified');
assert(primaryColor == null || brightness == null, 'Only one of primaryColor or brightness may be specified');
if (primaryColor != null) {
brightness = ThemeData.estimateBrightnessForColor(primaryColor);
}
// These are Material Design defaults, and are used to derive
// component Colors (with opacity) from base colors.
const int backgroundAlpha = 0x1f; // 12%
const int deleteIconAlpha = 0xde; // 87%
const int disabledAlpha = 0x0c; // 38% * 12% = 5%
const int selectAlpha = 0x3d; // 12% + 12% = 24%
const int textLabelAlpha = 0xde; // 87%
const EdgeInsetsGeometry padding = EdgeInsets.all(4.0);
primaryColor = primaryColor ?? (brightness == Brightness.light ? Colors.black : Colors.white);
final Color backgroundColor = primaryColor.withAlpha(backgroundAlpha);
final Color deleteIconColor = primaryColor.withAlpha(deleteIconAlpha);
final Color disabledColor = primaryColor.withAlpha(disabledAlpha);
final Color selectedColor = primaryColor.withAlpha(selectAlpha);
final Color secondarySelectedColor = secondaryColor.withAlpha(selectAlpha);
final TextStyle secondaryLabelStyle = labelStyle.copyWith(
color: secondaryColor.withAlpha(textLabelAlpha),
);
labelStyle = labelStyle.copyWith(color: primaryColor.withAlpha(textLabelAlpha));
return ChipThemeData(
backgroundColor: backgroundColor,
deleteIconColor: deleteIconColor,
disabledColor: disabledColor,
selectedColor: selectedColor,
secondarySelectedColor: secondarySelectedColor,
shadowColor: Colors.black,
selectedShadowColor: Colors.black,
showCheckmark: true,
padding: padding,
labelStyle: labelStyle,
secondaryLabelStyle: secondaryLabelStyle,
brightness: brightness,
elevation: 0.0,
pressElevation: 8.0,
);
}
/// Overrides the default for [ChipAttributes.color].
///
/// This property applies to [ActionChip], [Chip], [ChoiceChip],
/// [FilterChip], [InputChip], [RawChip].
final MaterialStateProperty<Color?>? color;
/// Overrides the default for [ChipAttributes.backgroundColor]
/// which is used for unselected, enabled chip backgrounds.
///
/// This property applies to [ActionChip], [Chip], [ChoiceChip],
/// [FilterChip], [InputChip], [RawChip].
final Color? backgroundColor;
/// Overrides the default for [DeletableChipAttributes.deleteIconColor].
///
/// This property applies to [Chip], [InputChip], [RawChip].
final Color? deleteIconColor;
/// Overrides the default for
/// [DisabledChipAttributes.disabledColor], the background color
/// which indicates that the chip is not enabled.
///
/// This property applies to [ActionChip], [ChoiceChip],
/// [FilterChip], [InputChip], and [RawChip].
final Color? disabledColor;
/// Overrides the default for
/// [SelectableChipAttributes.selectedColor], the background color
/// that indicates that the chip is selected.
///
/// This property applies to [ChoiceChip], [FilterChip],
/// [InputChip], [RawChip].
final Color? selectedColor;
/// Overrides the default for [ChoiceChip.selectedColor], the
/// background color that indicates that the chip is selected.
final Color? secondarySelectedColor;
/// Overrides the default for [ChipAttributes.shadowColor], the
/// Color of the chip's shadow when its elevation is greater than 0.
///
/// This property applies to [ActionChip], [Chip], [ChoiceChip],
/// [FilterChip], [InputChip], [RawChip].
final Color? shadowColor;
/// Overrides the default for [ChipAttributes.surfaceTintColor], the
/// Color of the chip's surface tint overlay when its elevation is
/// greater than 0.
///
/// This property applies to [ActionChip], [Chip], [ChoiceChip],
/// [FilterChip], [InputChip], [RawChip].
final Color? surfaceTintColor;
/// Overrides the default for
/// [SelectableChipAttributes.selectedShadowColor], the Color of the
/// chip's shadow when its elevation is greater than 0 and the chip
/// is selected.
///
/// This property applies to [ChoiceChip], [FilterChip],
/// [InputChip], [RawChip].
final Color? selectedShadowColor;
/// Overrides the default for
/// [CheckmarkableChipAttributes.showCheckmark], which indicates if
/// a check mark should be shown.
///
/// This property applies to [FilterChip], [InputChip], [RawChip].
final bool? showCheckmark;
/// Overrides the default for
/// [CheckmarkableChipAttributes.checkmarkColor].
///
/// This property applies to [FilterChip], [InputChip], [RawChip].
final Color? checkmarkColor;
/// Overrides the default for [ChipAttributes.labelPadding],
/// the padding around the chip's label widget.
///
/// This property applies to [ActionChip], [Chip], [ChoiceChip],
/// [FilterChip], [InputChip], [RawChip].
final EdgeInsetsGeometry? labelPadding;
/// Overrides the default for [ChipAttributes.padding],
/// the padding between the contents of the chip and the outside [shape].
///
/// This property applies to [ActionChip], [Chip], [ChoiceChip],
/// [FilterChip], [InputChip], [RawChip].
final EdgeInsetsGeometry? padding;
/// Overrides the default for [ChipAttributes.side],
/// the color and weight of the chip's outline.
///
/// This value is combined with [shape] to create a shape decorated with an
/// outline. If it is a [MaterialStateBorderSide],
/// [MaterialStateProperty.resolve] is used for the following
/// [MaterialState]s:
///
/// * [MaterialState.disabled].
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// * [MaterialState.pressed].
///
/// This property applies to [ActionChip], [Chip], [ChoiceChip],
/// [FilterChip], [InputChip], [RawChip].
final BorderSide? side;
/// Overrides the default for [ChipAttributes.shape],
/// the shape of border to draw around the chip.
///
/// This shape is combined with [side] to create a shape decorated with an
/// outline. If it is a [MaterialStateOutlinedBorder],
/// [MaterialStateProperty.resolve] is used for the following
/// [MaterialState]s:
///
/// * [MaterialState.disabled].
/// * [MaterialState.selected].
/// * [MaterialState.hovered].
/// * [MaterialState.focused].
/// * [MaterialState.pressed].
///
/// This property applies to [ActionChip], [Chip], [ChoiceChip],
/// [FilterChip], [InputChip], [RawChip].
final OutlinedBorder? shape;
/// Overrides the default for [ChipAttributes.labelStyle],
/// the style of the [DefaultTextStyle] that contains the
/// chip's label.
///
/// This only has an effect on label widgets that respect the
/// [DefaultTextStyle], such as [Text].
///
/// This property applies to [ActionChip], [Chip],
/// [FilterChip], [InputChip], [RawChip].
final TextStyle? labelStyle;
/// Overrides the default for [ChoiceChip.labelStyle],
/// the style of the [DefaultTextStyle] that contains the
/// chip's label.
///
/// This only has an effect on label widgets that respect the
/// [DefaultTextStyle], such as [Text].
final TextStyle? secondaryLabelStyle;
/// Overrides the default value for all chips which affects various base
/// material color choices in the chip rendering.
final Brightness? brightness;
/// Overrides the default for [ChipAttributes.elevation],
/// the elevation of the chip's [Material].
///
/// This property applies to [ActionChip], [Chip], [ChoiceChip],
/// [FilterChip], [InputChip], [RawChip].
final double? elevation;
/// Overrides the default for [TappableChipAttributes.pressElevation],
/// the elevation of the chip's [Material] during a "press" or tap down.
///
/// This property applies to [ActionChip], [InputChip], [RawChip].
final double? pressElevation;
/// Overrides the default for [ChipAttributes.iconTheme],
/// the theme used for all icons in the chip.
///
/// This property applies to [ActionChip], [Chip], [ChoiceChip],
/// [FilterChip], [InputChip], [RawChip].
final IconThemeData? iconTheme;
/// Overrides the default for [ChipAttributes.avatarBoxConstraints],
/// the size constraints for the avatar widget.
///
/// This property applies to [ActionChip], [Chip], [ChoiceChip],
/// [FilterChip], [InputChip], [RawChip].
final BoxConstraints? avatarBoxConstraints;
/// Overrides the default for [DeletableChipAttributes.deleteIconBoxConstraints].
/// the size constraints for the delete icon widget.
///
/// This property applies to [Chip], [FilterChip], [InputChip], [RawChip].
final BoxConstraints? deleteIconBoxConstraints;
/// Creates a copy of this object but with the given fields replaced with the
/// new values.
ChipThemeData copyWith({
MaterialStateProperty<Color?>? color,
Color? backgroundColor,
Color? deleteIconColor,
Color? disabledColor,
Color? selectedColor,
Color? secondarySelectedColor,
Color? shadowColor,
Color? surfaceTintColor,
Color? selectedShadowColor,
bool? showCheckmark,
Color? checkmarkColor,
EdgeInsetsGeometry? labelPadding,
EdgeInsetsGeometry? padding,
BorderSide? side,
OutlinedBorder? shape,
TextStyle? labelStyle,
TextStyle? secondaryLabelStyle,
Brightness? brightness,
double? elevation,
double? pressElevation,
IconThemeData? iconTheme,
BoxConstraints? avatarBoxConstraints,
BoxConstraints? deleteIconBoxConstraints,
}) {
return ChipThemeData(
color: color ?? this.color,
backgroundColor: backgroundColor ?? this.backgroundColor,
deleteIconColor: deleteIconColor ?? this.deleteIconColor,
disabledColor: disabledColor ?? this.disabledColor,
selectedColor: selectedColor ?? this.selectedColor,
secondarySelectedColor: secondarySelectedColor ?? this.secondarySelectedColor,
shadowColor: shadowColor ?? this.shadowColor,
surfaceTintColor: surfaceTintColor ?? this.surfaceTintColor,
selectedShadowColor: selectedShadowColor ?? this.selectedShadowColor,
showCheckmark: showCheckmark ?? this.showCheckmark,
checkmarkColor: checkmarkColor ?? this.checkmarkColor,
labelPadding: labelPadding ?? this.labelPadding,
padding: padding ?? this.padding,
side: side ?? this.side,
shape: shape ?? this.shape,
labelStyle: labelStyle ?? this.labelStyle,
secondaryLabelStyle: secondaryLabelStyle ?? this.secondaryLabelStyle,
brightness: brightness ?? this.brightness,
elevation: elevation ?? this.elevation,
pressElevation: pressElevation ?? this.pressElevation,
iconTheme: iconTheme ?? this.iconTheme,
avatarBoxConstraints: avatarBoxConstraints ?? this.avatarBoxConstraints,
deleteIconBoxConstraints: deleteIconBoxConstraints ?? this.deleteIconBoxConstraints,
);
}
/// Linearly interpolate between two chip themes.
///
/// {@macro dart.ui.shadow.lerp}
static ChipThemeData? lerp(ChipThemeData? a, ChipThemeData? b, double t) {
if (identical(a, b)) {
return a;
}
return ChipThemeData(
color: MaterialStateProperty.lerp<Color?>(a?.color, b?.color, t, Color.lerp),
backgroundColor: Color.lerp(a?.backgroundColor, b?.backgroundColor, t),
deleteIconColor: Color.lerp(a?.deleteIconColor, b?.deleteIconColor, t),
disabledColor: Color.lerp(a?.disabledColor, b?.disabledColor, t),
selectedColor: Color.lerp(a?.selectedColor, b?.selectedColor, t),
secondarySelectedColor: Color.lerp(a?.secondarySelectedColor, b?.secondarySelectedColor, t),
shadowColor: Color.lerp(a?.shadowColor, b?.shadowColor, t),
surfaceTintColor: Color.lerp(a?.surfaceTintColor, b?.surfaceTintColor, t),
selectedShadowColor: Color.lerp(a?.selectedShadowColor, b?.selectedShadowColor, t),
showCheckmark: t < 0.5 ? a?.showCheckmark ?? true : b?.showCheckmark ?? true,
checkmarkColor: Color.lerp(a?.checkmarkColor, b?.checkmarkColor, t),
labelPadding: EdgeInsetsGeometry.lerp(a?.labelPadding, b?.labelPadding, t),
padding: EdgeInsetsGeometry.lerp(a?.padding, b?.padding, t),
side: _lerpSides(a?.side, b?.side, t),
shape: _lerpShapes(a?.shape, b?.shape, t),
labelStyle: TextStyle.lerp(a?.labelStyle, b?.labelStyle, t),
secondaryLabelStyle: TextStyle.lerp(a?.secondaryLabelStyle, b?.secondaryLabelStyle, t),
brightness: t < 0.5 ? a?.brightness ?? Brightness.light : b?.brightness ?? Brightness.light,
elevation: lerpDouble(a?.elevation, b?.elevation, t),
pressElevation: lerpDouble(a?.pressElevation, b?.pressElevation, t),
iconTheme: a?.iconTheme != null || b?.iconTheme != null
? IconThemeData.lerp(a?.iconTheme, b?.iconTheme, t)
: null,
avatarBoxConstraints: BoxConstraints.lerp(a?.avatarBoxConstraints, b?.avatarBoxConstraints, t),
deleteIconBoxConstraints: BoxConstraints.lerp(a?.deleteIconBoxConstraints, b?.deleteIconBoxConstraints, t),
);
}
// Special case because BorderSide.lerp() doesn't support null arguments.
static BorderSide? _lerpSides(BorderSide? a, BorderSide? b, double t) {
if (a == null && b == null) {
return null;
}
if (a == null) {
return BorderSide.lerp(BorderSide(width: 0, color: b!.color.withAlpha(0)), b, t);
}
if (b == null) {
return BorderSide.lerp(BorderSide(width: 0, color: a.color.withAlpha(0)), a, t);
}
return BorderSide.lerp(a, b, t);
}
// TODO(perclasson): OutlinedBorder needs a lerp method - https://github.com/flutter/flutter/issues/60555.
static OutlinedBorder? _lerpShapes(OutlinedBorder? a, OutlinedBorder? b, double t) {
if (a == null && b == null) {
return null;
}
return ShapeBorder.lerp(a, b, t) as OutlinedBorder?;
}
@override
int get hashCode => Object.hashAll(<Object?>[
color,
backgroundColor,
deleteIconColor,
disabledColor,
selectedColor,
secondarySelectedColor,
shadowColor,
surfaceTintColor,
selectedShadowColor,
showCheckmark,
checkmarkColor,
labelPadding,
padding,
side,
shape,
labelStyle,
secondaryLabelStyle,
brightness,
elevation,
pressElevation,
iconTheme,
avatarBoxConstraints,
deleteIconBoxConstraints,
]);
@override
bool operator ==(Object other) {
if (identical(this, other)) {
return true;
}
if (other.runtimeType != runtimeType) {
return false;
}
return other is ChipThemeData
&& other.color == color
&& other.backgroundColor == backgroundColor
&& other.deleteIconColor == deleteIconColor
&& other.disabledColor == disabledColor
&& other.selectedColor == selectedColor
&& other.secondarySelectedColor == secondarySelectedColor
&& other.shadowColor == shadowColor
&& other.surfaceTintColor == surfaceTintColor
&& other.selectedShadowColor == selectedShadowColor
&& other.showCheckmark == showCheckmark
&& other.checkmarkColor == checkmarkColor
&& other.labelPadding == labelPadding
&& other.padding == padding
&& other.side == side
&& other.shape == shape
&& other.labelStyle == labelStyle
&& other.secondaryLabelStyle == secondaryLabelStyle
&& other.brightness == brightness
&& other.elevation == elevation
&& other.pressElevation == pressElevation
&& other.iconTheme == iconTheme
&& other.avatarBoxConstraints == avatarBoxConstraints
&& other.deleteIconBoxConstraints == deleteIconBoxConstraints;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<MaterialStateProperty<Color?>>('color', color, defaultValue: null));
properties.add(ColorProperty('backgroundColor', backgroundColor, defaultValue: null));
properties.add(ColorProperty('deleteIconColor', deleteIconColor, defaultValue: null));
properties.add(ColorProperty('disabledColor', disabledColor, defaultValue: null));
properties.add(ColorProperty('selectedColor', selectedColor, defaultValue: null));
properties.add(ColorProperty('secondarySelectedColor', secondarySelectedColor, defaultValue: null));
properties.add(ColorProperty('shadowColor', shadowColor, defaultValue: null));
properties.add(ColorProperty('surfaceTintColor', surfaceTintColor, defaultValue: null));
properties.add(ColorProperty('selectedShadowColor', selectedShadowColor, defaultValue: null));
properties.add(DiagnosticsProperty<bool>('showCheckmark', showCheckmark, defaultValue: null));
properties.add(ColorProperty('checkMarkColor', checkmarkColor, defaultValue: null));
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('labelPadding', labelPadding, defaultValue: null));
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('padding', padding, defaultValue: null));
properties.add(DiagnosticsProperty<BorderSide>('side', side, defaultValue: null));
properties.add(DiagnosticsProperty<ShapeBorder>('shape', shape, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('labelStyle', labelStyle, defaultValue: null));
properties.add(DiagnosticsProperty<TextStyle>('secondaryLabelStyle', secondaryLabelStyle, defaultValue: null));
properties.add(EnumProperty<Brightness>('brightness', brightness, defaultValue: null));
properties.add(DoubleProperty('elevation', elevation, defaultValue: null));
properties.add(DoubleProperty('pressElevation', pressElevation, defaultValue: null));
properties.add(DiagnosticsProperty<IconThemeData>('iconTheme', iconTheme, defaultValue: null));
properties.add(DiagnosticsProperty<BoxConstraints>('avatarBoxConstraints', avatarBoxConstraints, defaultValue: null));
properties.add(DiagnosticsProperty<BoxConstraints>('deleteIconBoxConstraints', deleteIconBoxConstraints, defaultValue: null));
}
}
| flutter/packages/flutter/lib/src/material/chip_theme.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/chip_theme.dart",
"repo_id": "flutter",
"token_count": 8246
} | 650 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart';
import 'colors.dart';
import 'constants.dart';
import 'text_button.dart';
import 'theme.dart';
const TextStyle _kToolbarButtonFontStyle = TextStyle(
inherit: false,
fontSize: 14.0,
letterSpacing: -0.15,
fontWeight: FontWeight.w400,
);
const EdgeInsets _kToolbarButtonPadding = EdgeInsets.fromLTRB(
20.0,
0.0,
20.0,
3.0,
);
/// A [TextButton] for the Material desktop text selection toolbar.
class DesktopTextSelectionToolbarButton extends StatelessWidget {
/// Creates an instance of DesktopTextSelectionToolbarButton.
const DesktopTextSelectionToolbarButton({
super.key,
required this.onPressed,
required this.child,
});
/// Create an instance of [DesktopTextSelectionToolbarButton] whose child is
/// a [Text] widget in the style of the Material text selection toolbar.
DesktopTextSelectionToolbarButton.text({
super.key,
required BuildContext context,
required this.onPressed,
required String text,
}) : child = Text(
text,
overflow: TextOverflow.ellipsis,
style: _kToolbarButtonFontStyle.copyWith(
color: Theme.of(context).colorScheme.brightness == Brightness.dark
? Colors.white
: Colors.black87,
),
);
/// {@macro flutter.material.TextSelectionToolbarTextButton.onPressed}
final VoidCallback? onPressed;
/// {@macro flutter.material.TextSelectionToolbarTextButton.child}
final Widget child;
@override
Widget build(BuildContext context) {
// TODO(hansmuller): Should be colorScheme.onSurface
final ThemeData theme = Theme.of(context);
final bool isDark = theme.colorScheme.brightness == Brightness.dark;
final Color foregroundColor = isDark ? Colors.white : Colors.black87;
return SizedBox(
width: double.infinity,
child: TextButton(
style: TextButton.styleFrom(
alignment: Alignment.centerLeft,
enabledMouseCursor: SystemMouseCursors.basic,
disabledMouseCursor: SystemMouseCursors.basic,
foregroundColor: foregroundColor,
shape: const RoundedRectangleBorder(),
minimumSize: const Size(kMinInteractiveDimension, 36.0),
padding: _kToolbarButtonPadding,
),
onPressed: onPressed,
child: child,
),
);
}
}
| flutter/packages/flutter/lib/src/material/desktop_text_selection_toolbar_button.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/desktop_text_selection_toolbar_button.dart",
"repo_id": "flutter",
"token_count": 941
} | 651 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'expansion_tile_theme.dart';
import 'icons.dart';
import 'list_tile.dart';
import 'list_tile_theme.dart';
import 'material.dart';
import 'material_localizations.dart';
import 'theme.dart';
import 'theme_data.dart';
const Duration _kExpand = Duration(milliseconds: 200);
/// Enables control over a single [ExpansionTile]'s expanded/collapsed state.
///
/// It can be useful to expand or collapse an [ExpansionTile]
/// programmatically, for example to reconfigure an existing expansion
/// tile based on a system event. To do so, create an [ExpansionTile]
/// with an [ExpansionTileController] that's owned by a stateful widget
/// or look up the tile's automatically created [ExpansionTileController]
/// with [ExpansionTileController.of]
///
/// The controller's [expand] and [collapse] methods cause the
/// the [ExpansionTile] to rebuild, so they may not be called from
/// a build method.
class ExpansionTileController {
/// Create a controller to be used with [ExpansionTile.controller].
ExpansionTileController();
_ExpansionTileState? _state;
/// Whether the [ExpansionTile] built with this controller is in expanded state.
///
/// This property doesn't take the animation into account. It reports `true`
/// even if the expansion animation is not completed.
///
/// See also:
///
/// * [expand], which expands the [ExpansionTile].
/// * [collapse], which collapses the [ExpansionTile].
/// * [ExpansionTile.controller] to create an ExpansionTile with a controller.
bool get isExpanded {
assert(_state != null);
return _state!._isExpanded;
}
/// Expands the [ExpansionTile] that was built with this controller;
///
/// Normally the tile is expanded automatically when the user taps on the header.
/// It is sometimes useful to trigger the expansion programmatically due
/// to external changes.
///
/// If the tile is already in the expanded state (see [isExpanded]), calling
/// this method has no effect.
///
/// Calling this method may cause the [ExpansionTile] to rebuild, so it may
/// not be called from a build method.
///
/// Calling this method will trigger an [ExpansionTile.onExpansionChanged] callback.
///
/// See also:
///
/// * [collapse], which collapses the tile.
/// * [isExpanded] to check whether the tile is expanded.
/// * [ExpansionTile.controller] to create an ExpansionTile with a controller.
void expand() {
assert(_state != null);
if (!isExpanded) {
_state!._toggleExpansion();
}
}
/// Collapses the [ExpansionTile] that was built with this controller.
///
/// Normally the tile is collapsed automatically when the user taps on the header.
/// It can be useful sometimes to trigger the collapse programmatically due
/// to some external changes.
///
/// If the tile is already in the collapsed state (see [isExpanded]), calling
/// this method has no effect.
///
/// Calling this method may cause the [ExpansionTile] to rebuild, so it may
/// not be called from a build method.
///
/// Calling this method will trigger an [ExpansionTile.onExpansionChanged] callback.
///
/// See also:
///
/// * [expand], which expands the tile.
/// * [isExpanded] to check whether the tile is expanded.
/// * [ExpansionTile.controller] to create an ExpansionTile with a controller.
void collapse() {
assert(_state != null);
if (isExpanded) {
_state!._toggleExpansion();
}
}
/// Finds the [ExpansionTileController] for the closest [ExpansionTile] instance
/// that encloses the given context.
///
/// If no [ExpansionTile] encloses the given context, calling this
/// method will cause an assert in debug mode, and throw an
/// exception in release mode.
///
/// To return null if there is no [ExpansionTile] use [maybeOf] instead.
///
/// {@tool dartpad}
/// Typical usage of the [ExpansionTileController.of] function is to call it from within the
/// `build` method of a descendant of an [ExpansionTile].
///
/// When the [ExpansionTile] is actually created in the same `build`
/// function as the callback that refers to the controller, then the
/// `context` argument to the `build` function can't be used to find
/// the [ExpansionTileController] (since it's "above" the widget
/// being returned in the widget tree). In cases like that you can
/// add a [Builder] widget, which provides a new scope with a
/// [BuildContext] that is "under" the [ExpansionTile]:
///
/// ** See code in examples/api/lib/material/expansion_tile/expansion_tile.1.dart **
/// {@end-tool}
///
/// A more efficient solution is to split your build function into
/// several widgets. This introduces a new context from which you
/// can obtain the [ExpansionTileController]. With this approach you
/// would have an outer widget that creates the [ExpansionTile]
/// populated by instances of your new inner widgets, and then in
/// these inner widgets you would use [ExpansionTileController.of].
static ExpansionTileController of(BuildContext context) {
final _ExpansionTileState? result = context.findAncestorStateOfType<_ExpansionTileState>();
if (result != null) {
return result._tileController;
}
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary(
'ExpansionTileController.of() called with a context that does not contain a ExpansionTile.',
),
ErrorDescription(
'No ExpansionTile ancestor could be found starting from the context that was passed to ExpansionTileController.of(). '
'This usually happens when the context provided is from the same StatefulWidget as that '
'whose build function actually creates the ExpansionTile widget being sought.',
),
ErrorHint(
'There are several ways to avoid this problem. The simplest is to use a Builder to get a '
'context that is "under" the ExpansionTile. For an example of this, please see the '
'documentation for ExpansionTileController.of():\n'
' https://api.flutter.dev/flutter/material/ExpansionTile/of.html',
),
ErrorHint(
'A more efficient solution is to split your build function into several widgets. This '
'introduces a new context from which you can obtain the ExpansionTile. In this solution, '
'you would have an outer widget that creates the ExpansionTile populated by instances of '
'your new inner widgets, and then in these inner widgets you would use ExpansionTileController.of().\n'
'An other solution is assign a GlobalKey to the ExpansionTile, '
'then use the key.currentState property to obtain the ExpansionTile rather than '
'using the ExpansionTileController.of() function.',
),
context.describeElement('The context used was'),
]);
}
/// Finds the [ExpansionTile] from the closest instance of this class that
/// encloses the given context and returns its [ExpansionTileController].
///
/// If no [ExpansionTile] encloses the given context then return null.
/// To throw an exception instead, use [of] instead of this function.
///
/// See also:
///
/// * [of], a similar function to this one that throws if no [ExpansionTile]
/// encloses the given context. Also includes some sample code in its
/// documentation.
static ExpansionTileController? maybeOf(BuildContext context) {
return context.findAncestorStateOfType<_ExpansionTileState>()?._tileController;
}
}
/// A single-line [ListTile] with an expansion arrow icon that expands or collapses
/// the tile to reveal or hide the [children].
///
/// This widget is typically used with [ListView] to create an "expand /
/// collapse" list entry. When used with scrolling widgets like [ListView], a
/// unique [PageStorageKey] must be specified as the [key], to enable the
/// [ExpansionTile] to save and restore its expanded state when it is scrolled
/// in and out of view.
///
/// This class overrides the [ListTileThemeData.iconColor] and [ListTileThemeData.textColor]
/// theme properties for its [ListTile]. These colors animate between values when
/// the tile is expanded and collapsed: between [iconColor], [collapsedIconColor] and
/// between [textColor] and [collapsedTextColor].
///
/// The expansion arrow icon is shown on the right by default in left-to-right languages
/// (i.e. the trailing edge). This can be changed using [controlAffinity]. This maps
/// to the [leading] and [trailing] properties of [ExpansionTile].
///
/// {@tool dartpad}
/// This example demonstrates how the [ExpansionTile] icon's location and appearance
/// can be customized.
///
/// ** See code in examples/api/lib/material/expansion_tile/expansion_tile.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example demonstrates how an [ExpansionTileController] can be used to
/// programmatically expand or collapse an [ExpansionTile].
///
/// ** See code in examples/api/lib/material/expansion_tile/expansion_tile.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [ListTile], useful for creating expansion tile [children] when the
/// expansion tile represents a sublist.
/// * The "Expand and collapse" section of
/// <https://material.io/components/lists#types>
class ExpansionTile extends StatefulWidget {
/// Creates a single-line [ListTile] with an expansion arrow icon that expands or collapses
/// the tile to reveal or hide the [children]. The [initiallyExpanded] property must
/// be non-null.
const ExpansionTile({
super.key,
this.leading,
required this.title,
this.subtitle,
this.onExpansionChanged,
this.children = const <Widget>[],
this.trailing,
this.initiallyExpanded = false,
this.maintainState = false,
this.tilePadding,
this.expandedCrossAxisAlignment,
this.expandedAlignment,
this.childrenPadding,
this.backgroundColor,
this.collapsedBackgroundColor,
this.textColor,
this.collapsedTextColor,
this.iconColor,
this.collapsedIconColor,
this.shape,
this.collapsedShape,
this.clipBehavior,
this.controlAffinity,
this.controller,
this.dense,
this.visualDensity,
this.minTileHeight,
this.enableFeedback = true,
this.enabled = true,
this.expansionAnimationStyle,
}) : assert(
expandedCrossAxisAlignment != CrossAxisAlignment.baseline,
'CrossAxisAlignment.baseline is not supported since the expanded children '
'are aligned in a column, not a row. Try to use another constant.',
);
/// A widget to display before the title.
///
/// Typically a [CircleAvatar] widget.
///
/// Depending on the value of [controlAffinity], the [leading] widget
/// may replace the rotating expansion arrow icon.
final Widget? leading;
/// The primary content of the list item.
///
/// Typically a [Text] widget.
final Widget title;
/// Additional content displayed below the title.
///
/// Typically a [Text] widget.
final Widget? subtitle;
/// Called when the tile expands or collapses.
///
/// When the tile starts expanding, this function is called with the value
/// true. When the tile starts collapsing, this function is called with
/// the value false.
final ValueChanged<bool>? onExpansionChanged;
/// The widgets that are displayed when the tile expands.
///
/// Typically [ListTile] widgets.
final List<Widget> children;
/// The color to display behind the sublist when expanded.
///
/// If this property is null then [ExpansionTileThemeData.backgroundColor] is used. If that
/// is also null then Colors.transparent is used.
///
/// See also:
///
/// * [ExpansionTileTheme.of], which returns the nearest [ExpansionTileTheme]'s
/// [ExpansionTileThemeData].
final Color? backgroundColor;
/// When not null, defines the background color of tile when the sublist is collapsed.
///
/// If this property is null then [ExpansionTileThemeData.collapsedBackgroundColor] is used.
/// If that is also null then Colors.transparent is used.
///
/// See also:
///
/// * [ExpansionTileTheme.of], which returns the nearest [ExpansionTileTheme]'s
/// [ExpansionTileThemeData].
final Color? collapsedBackgroundColor;
/// A widget to display after the title.
///
/// Depending on the value of [controlAffinity], the [trailing] widget
/// may replace the rotating expansion arrow icon.
final Widget? trailing;
/// Specifies if the list tile is initially expanded (true) or collapsed (false, the default).
final bool initiallyExpanded;
/// Specifies whether the state of the children is maintained when the tile expands and collapses.
///
/// When true, the children are kept in the tree while the tile is collapsed.
/// When false (default), the children are removed from the tree when the tile is
/// collapsed and recreated upon expansion.
final bool maintainState;
/// Specifies padding for the [ListTile].
///
/// Analogous to [ListTile.contentPadding], this property defines the insets for
/// the [leading], [title], [subtitle] and [trailing] widgets. It does not inset
/// the expanded [children] widgets.
///
/// If this property is null then [ExpansionTileThemeData.tilePadding] is used. If that
/// is also null then the tile's padding is `EdgeInsets.symmetric(horizontal: 16.0)`.
///
/// See also:
///
/// * [ExpansionTileTheme.of], which returns the nearest [ExpansionTileTheme]'s
/// [ExpansionTileThemeData].
final EdgeInsetsGeometry? tilePadding;
/// Specifies the alignment of [children], which are arranged in a column when
/// the tile is expanded.
///
/// The internals of the expanded tile make use of a [Column] widget for
/// [children], and [Align] widget to align the column. The [expandedAlignment]
/// parameter is passed directly into the [Align].
///
/// Modifying this property controls the alignment of the column within the
/// expanded tile, not the alignment of [children] widgets within the column.
/// To align each child within [children], see [expandedCrossAxisAlignment].
///
/// The width of the column is the width of the widest child widget in [children].
///
/// If this property is null then [ExpansionTileThemeData.expandedAlignment]is used. If that
/// is also null then the value of [expandedAlignment] is [Alignment.center].
///
/// See also:
///
/// * [ExpansionTileTheme.of], which returns the nearest [ExpansionTileTheme]'s
/// [ExpansionTileThemeData].
final Alignment? expandedAlignment;
/// Specifies the alignment of each child within [children] when the tile is expanded.
///
/// The internals of the expanded tile make use of a [Column] widget for
/// [children], and the `crossAxisAlignment` parameter is passed directly into
/// the [Column].
///
/// Modifying this property controls the cross axis alignment of each child
/// within its [Column]. The width of the [Column] that houses [children] will
/// be the same as the widest child widget in [children]. The width of the
/// [Column] might not be equal to the width of the expanded tile.
///
/// To align the [Column] along the expanded tile, use the [expandedAlignment]
/// property instead.
///
/// When the value is null, the value of [expandedCrossAxisAlignment] is
/// [CrossAxisAlignment.center].
final CrossAxisAlignment? expandedCrossAxisAlignment;
/// Specifies padding for [children].
///
/// If this property is null then [ExpansionTileThemeData.childrenPadding] is used. If that
/// is also null then the value of [childrenPadding] is [EdgeInsets.zero].
///
/// See also:
///
/// * [ExpansionTileTheme.of], which returns the nearest [ExpansionTileTheme]'s
/// [ExpansionTileThemeData].
final EdgeInsetsGeometry? childrenPadding;
/// The icon color of tile's expansion arrow icon when the sublist is expanded.
///
/// Used to override to the [ListTileThemeData.iconColor].
///
/// If this property is null then [ExpansionTileThemeData.iconColor] is used. If that
/// is also null then the value of [ColorScheme.primary] is used.
///
/// See also:
///
/// * [ExpansionTileTheme.of], which returns the nearest [ExpansionTileTheme]'s
/// [ExpansionTileThemeData].
final Color? iconColor;
/// The icon color of tile's expansion arrow icon when the sublist is collapsed.
///
/// Used to override to the [ListTileThemeData.iconColor].
///
/// If this property is null then [ExpansionTileThemeData.collapsedIconColor] is used. If that
/// is also null and [ThemeData.useMaterial3] is true, [ColorScheme.onSurface] is used. Otherwise,
/// defaults to [ThemeData.unselectedWidgetColor] color.
///
/// See also:
///
/// * [ExpansionTileTheme.of], which returns the nearest [ExpansionTileTheme]'s
/// [ExpansionTileThemeData].
final Color? collapsedIconColor;
/// The color of the tile's titles when the sublist is expanded.
///
/// Used to override to the [ListTileThemeData.textColor].
///
/// If this property is null then [ExpansionTileThemeData.textColor] is used. If that
/// is also null then and [ThemeData.useMaterial3] is true, color of the [TextTheme.bodyLarge]
/// will be used for the [title] and [subtitle]. Otherwise, defaults to [ColorScheme.primary] color.
///
/// See also:
///
/// * [ExpansionTileTheme.of], which returns the nearest [ExpansionTileTheme]'s
/// [ExpansionTileThemeData].
final Color? textColor;
/// The color of the tile's titles when the sublist is collapsed.
///
/// Used to override to the [ListTileThemeData.textColor].
///
/// If this property is null then [ExpansionTileThemeData.collapsedTextColor] is used.
/// If that is also null and [ThemeData.useMaterial3] is true, color of the
/// [TextTheme.bodyLarge] will be used for the [title] and [subtitle]. Otherwise,
/// defaults to color of the [TextTheme.titleMedium].
///
/// See also:
///
/// * [ExpansionTileTheme.of], which returns the nearest [ExpansionTileTheme]'s
/// [ExpansionTileThemeData].
final Color? collapsedTextColor;
/// The tile's border shape when the sublist is expanded.
///
/// If this property is null, the [ExpansionTileThemeData.shape] is used. If that
/// is also null, a [Border] with vertical sides default to [ThemeData.dividerColor] is used
///
/// See also:
///
/// * [ExpansionTileTheme.of], which returns the nearest [ExpansionTileTheme]'s
/// [ExpansionTileThemeData].
final ShapeBorder? shape;
/// The tile's border shape when the sublist is collapsed.
///
/// If this property is null, the [ExpansionTileThemeData.collapsedShape] is used. If that
/// is also null, a [Border] with vertical sides default to Color [Colors.transparent] is used
///
/// See also:
///
/// * [ExpansionTileTheme.of], which returns the nearest [ExpansionTileTheme]'s
/// [ExpansionTileThemeData].
final ShapeBorder? collapsedShape;
/// {@macro flutter.material.Material.clipBehavior}
///
/// If this is not null and a custom collapsed or expanded shape is provided,
/// the value of [clipBehavior] will be used to clip the expansion tile.
///
/// If this property is null, the [ExpansionTileThemeData.clipBehavior] is used. If that
/// is also null, defaults to [Clip.antiAlias].
///
/// See also:
///
/// * [ExpansionTileTheme.of], which returns the nearest [ExpansionTileTheme]'s
/// [ExpansionTileThemeData].
final Clip? clipBehavior;
/// Typically used to force the expansion arrow icon to the tile's leading or trailing edge.
///
/// By default, the value of [controlAffinity] is [ListTileControlAffinity.platform],
/// which means that the expansion arrow icon will appear on the tile's trailing edge.
final ListTileControlAffinity? controlAffinity;
/// If provided, the controller can be used to expand and collapse tiles.
///
/// In cases were control over the tile's state is needed from a callback triggered
/// by a widget within the tile, [ExpansionTileController.of] may be more convenient
/// than supplying a controller.
final ExpansionTileController? controller;
/// {@macro flutter.material.ListTile.dense}
final bool? dense;
/// Defines how compact the expansion tile's layout will be.
///
/// {@macro flutter.material.themedata.visualDensity}
final VisualDensity? visualDensity;
/// {@macro flutter.material.ListTile.minTileHeight}
final double? minTileHeight;
/// {@macro flutter.material.ListTile.enableFeedback}
final bool? enableFeedback;
/// Whether this expansion tile is interactive.
///
/// If false, the internal [ListTile] will be disabled, changing its
/// appearance according to the theme and disabling user interaction.
///
/// Even if disabled, the expansion can still be toggled programmatically
/// through an [ExpansionTileController].
final bool enabled;
/// Used to override the expansion animation curve and duration.
///
/// If [AnimationStyle.duration] is provided, it will be used to override
/// the expansion animation duration. If it is null, then [AnimationStyle.duration]
/// from the [ExpansionTileThemeData.expansionAnimationStyle] will be used.
/// Otherwise, defaults to 200ms.
///
/// If [AnimationStyle.curve] is provided, it will be used to override
/// the expansion animation curve. If it is null, then [AnimationStyle.curve]
/// from the [ExpansionTileThemeData.expansionAnimationStyle] will be used.
/// Otherwise, defaults to [Curves.easeIn].
///
/// To disable the theme animation, use [AnimationStyle.noAnimation].
///
/// {@tool dartpad}
/// This sample showcases how to override the [ExpansionTile] expansion
/// animation curve and duration using [AnimationStyle].
///
/// ** See code in examples/api/lib/material/expansion_tile/expansion_tile.2.dart **
/// {@end-tool}
final AnimationStyle? expansionAnimationStyle;
@override
State<ExpansionTile> createState() => _ExpansionTileState();
}
class _ExpansionTileState extends State<ExpansionTile> with SingleTickerProviderStateMixin {
static final Animatable<double> _easeOutTween = CurveTween(curve: Curves.easeOut);
static final Animatable<double> _easeInTween = CurveTween(curve: Curves.easeIn);
static final Animatable<double> _halfTween = Tween<double>(begin: 0.0, end: 0.5);
final ShapeBorderTween _borderTween = ShapeBorderTween();
final ColorTween _headerColorTween = ColorTween();
final ColorTween _iconColorTween = ColorTween();
final ColorTween _backgroundColorTween = ColorTween();
final CurveTween _heightFactorTween = CurveTween(curve: Curves.easeIn);
late AnimationController _animationController;
late Animation<double> _iconTurns;
late Animation<double> _heightFactor;
late Animation<ShapeBorder?> _border;
late Animation<Color?> _headerColor;
late Animation<Color?> _iconColor;
late Animation<Color?> _backgroundColor;
bool _isExpanded = false;
late ExpansionTileController _tileController;
@override
void initState() {
super.initState();
_animationController = AnimationController(duration: _kExpand, vsync: this);
_heightFactor = _animationController.drive(_heightFactorTween);
_iconTurns = _animationController.drive(_halfTween.chain(_easeInTween));
_border = _animationController.drive(_borderTween.chain(_easeOutTween));
_headerColor = _animationController.drive(_headerColorTween.chain(_easeInTween));
_iconColor = _animationController.drive(_iconColorTween.chain(_easeInTween));
_backgroundColor = _animationController.drive(_backgroundColorTween.chain(_easeOutTween));
_isExpanded = PageStorage.maybeOf(context)?.readState(context) as bool? ?? widget.initiallyExpanded;
if (_isExpanded) {
_animationController.value = 1.0;
}
assert(widget.controller?._state == null);
_tileController = widget.controller ?? ExpansionTileController();
_tileController._state = this;
}
@override
void dispose() {
_tileController._state = null;
_animationController.dispose();
super.dispose();
}
void _toggleExpansion() {
final TextDirection textDirection = WidgetsLocalizations.of(context).textDirection;
final MaterialLocalizations localizations = MaterialLocalizations.of(context);
final String stateHint = _isExpanded ? localizations.expandedHint : localizations.collapsedHint;
setState(() {
_isExpanded = !_isExpanded;
if (_isExpanded) {
_animationController.forward();
} else {
_animationController.reverse().then<void>((void value) {
if (!mounted) {
return;
}
setState(() {
// Rebuild without widget.children.
});
});
}
PageStorage.maybeOf(context)?.writeState(context, _isExpanded);
});
widget.onExpansionChanged?.call(_isExpanded);
SemanticsService.announce(stateHint, textDirection);
}
void _handleTap() {
_toggleExpansion();
}
// Platform or null affinity defaults to trailing.
ListTileControlAffinity _effectiveAffinity(ListTileControlAffinity? affinity) {
switch (affinity ?? ListTileControlAffinity.trailing) {
case ListTileControlAffinity.leading:
return ListTileControlAffinity.leading;
case ListTileControlAffinity.trailing:
case ListTileControlAffinity.platform:
return ListTileControlAffinity.trailing;
}
}
Widget? _buildIcon(BuildContext context) {
return RotationTransition(
turns: _iconTurns,
child: const Icon(Icons.expand_more),
);
}
Widget? _buildLeadingIcon(BuildContext context) {
if (_effectiveAffinity(widget.controlAffinity) != ListTileControlAffinity.leading) {
return null;
}
return _buildIcon(context);
}
Widget? _buildTrailingIcon(BuildContext context) {
if (_effectiveAffinity(widget.controlAffinity) != ListTileControlAffinity.trailing) {
return null;
}
return _buildIcon(context);
}
Widget _buildChildren(BuildContext context, Widget? child) {
final ThemeData theme = Theme.of(context);
final ExpansionTileThemeData expansionTileTheme = ExpansionTileTheme.of(context);
final Color backgroundColor = _backgroundColor.value ?? expansionTileTheme.backgroundColor ?? Colors.transparent;
final ShapeBorder expansionTileBorder = _border.value ?? const Border(
top: BorderSide(color: Colors.transparent),
bottom: BorderSide(color: Colors.transparent),
);
final Clip clipBehavior = widget.clipBehavior ?? expansionTileTheme.clipBehavior ?? Clip.antiAlias;
final MaterialLocalizations localizations = MaterialLocalizations.of(context);
final String onTapHint = _isExpanded
? localizations.expansionTileExpandedTapHint
: localizations.expansionTileCollapsedTapHint;
String? semanticsHint;
switch (theme.platform) {
case TargetPlatform.iOS:
case TargetPlatform.macOS:
semanticsHint = _isExpanded
? '${localizations.collapsedHint}\n ${localizations.expansionTileExpandedHint}'
: '${localizations.expandedHint}\n ${localizations.expansionTileCollapsedHint}';
case TargetPlatform.android:
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
break;
}
final Decoration decoration = ShapeDecoration(
color: backgroundColor,
shape: expansionTileBorder,
);
final Widget tile = Padding(
padding: decoration.padding,
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Semantics(
hint: semanticsHint,
onTapHint: onTapHint,
child: ListTileTheme.merge(
iconColor: _iconColor.value ?? expansionTileTheme.iconColor,
textColor: _headerColor.value,
child: ListTile(
enabled: widget.enabled,
onTap: _handleTap,
dense: widget.dense,
visualDensity: widget.visualDensity,
enableFeedback: widget.enableFeedback,
contentPadding: widget.tilePadding ?? expansionTileTheme.tilePadding,
leading: widget.leading ?? _buildLeadingIcon(context),
title: widget.title,
subtitle: widget.subtitle,
trailing: widget.trailing ?? _buildTrailingIcon(context),
minTileHeight: widget.minTileHeight,
),
),
),
ClipRect(
child: Align(
alignment: widget.expandedAlignment
?? expansionTileTheme.expandedAlignment
?? Alignment.center,
heightFactor: _heightFactor.value,
child: child,
),
),
],
),
);
final bool isShapeProvided = widget.shape != null || expansionTileTheme.shape != null
|| widget.collapsedShape != null || expansionTileTheme.collapsedShape != null;
if (isShapeProvided) {
return Material(
clipBehavior: clipBehavior,
color: backgroundColor,
shape: expansionTileBorder,
child: tile,
);
}
return DecoratedBox(
decoration: decoration,
child: tile,
);
}
@override
void didUpdateWidget(covariant ExpansionTile oldWidget) {
super.didUpdateWidget(oldWidget);
final ThemeData theme = Theme.of(context);
final ExpansionTileThemeData expansionTileTheme = ExpansionTileTheme.of(context);
final ExpansionTileThemeData defaults = theme.useMaterial3
? _ExpansionTileDefaultsM3(context)
: _ExpansionTileDefaultsM2(context);
if (widget.collapsedShape != oldWidget.collapsedShape
|| widget.shape != oldWidget.shape) {
_updateShapeBorder(expansionTileTheme, theme);
}
if (widget.collapsedTextColor != oldWidget.collapsedTextColor
|| widget.textColor != oldWidget.textColor) {
_updateHeaderColor(expansionTileTheme, defaults);
}
if (widget.collapsedIconColor != oldWidget.collapsedIconColor
|| widget.iconColor != oldWidget.iconColor) {
_updateIconColor(expansionTileTheme, defaults);
}
if (widget.backgroundColor != oldWidget.backgroundColor
|| widget.collapsedBackgroundColor != oldWidget.collapsedBackgroundColor) {
_updateBackgroundColor(expansionTileTheme);
}
if (widget.expansionAnimationStyle != oldWidget.expansionAnimationStyle) {
_updateAnimationDuration(expansionTileTheme);
_updateHeightFactorCurve(expansionTileTheme);
}
}
@override
void didChangeDependencies() {
final ThemeData theme = Theme.of(context);
final ExpansionTileThemeData expansionTileTheme = ExpansionTileTheme.of(context);
final ExpansionTileThemeData defaults = theme.useMaterial3
? _ExpansionTileDefaultsM3(context)
: _ExpansionTileDefaultsM2(context);
_updateAnimationDuration(expansionTileTheme);
_updateShapeBorder(expansionTileTheme, theme);
_updateHeaderColor(expansionTileTheme, defaults);
_updateIconColor(expansionTileTheme, defaults);
_updateBackgroundColor(expansionTileTheme);
_updateHeightFactorCurve(expansionTileTheme);
super.didChangeDependencies();
}
void _updateAnimationDuration(ExpansionTileThemeData expansionTileTheme) {
_animationController.duration = widget.expansionAnimationStyle?.duration
?? expansionTileTheme.expansionAnimationStyle?.duration
?? _kExpand;
}
void _updateShapeBorder(ExpansionTileThemeData expansionTileTheme, ThemeData theme) {
_borderTween
..begin = widget.collapsedShape
?? expansionTileTheme.collapsedShape
?? const Border(
top: BorderSide(color: Colors.transparent),
bottom: BorderSide(color: Colors.transparent),
)
..end = widget.shape
?? expansionTileTheme.shape
?? Border(
top: BorderSide(color: theme.dividerColor),
bottom: BorderSide(color: theme.dividerColor),
);
}
void _updateHeaderColor(ExpansionTileThemeData expansionTileTheme, ExpansionTileThemeData defaults) {
_headerColorTween
..begin = widget.collapsedTextColor
?? expansionTileTheme.collapsedTextColor
?? defaults.collapsedTextColor
..end = widget.textColor ?? expansionTileTheme.textColor ?? defaults.textColor;
}
void _updateIconColor(ExpansionTileThemeData expansionTileTheme, ExpansionTileThemeData defaults) {
_iconColorTween
..begin = widget.collapsedIconColor
?? expansionTileTheme.collapsedIconColor
?? defaults.collapsedIconColor
..end = widget.iconColor ?? expansionTileTheme.iconColor ?? defaults.iconColor;
}
void _updateBackgroundColor(ExpansionTileThemeData expansionTileTheme) {
_backgroundColorTween
..begin = widget.collapsedBackgroundColor ?? expansionTileTheme.collapsedBackgroundColor
..end = widget.backgroundColor ?? expansionTileTheme.backgroundColor;
}
void _updateHeightFactorCurve(ExpansionTileThemeData expansionTileTheme) {
_heightFactorTween.curve = widget.expansionAnimationStyle?.curve
?? expansionTileTheme.expansionAnimationStyle?.curve
?? Curves.easeIn;
}
@override
Widget build(BuildContext context) {
final ExpansionTileThemeData expansionTileTheme = ExpansionTileTheme.of(context);
final bool closed = !_isExpanded && _animationController.isDismissed;
final bool shouldRemoveChildren = closed && !widget.maintainState;
final Widget result = Offstage(
offstage: closed,
child: TickerMode(
enabled: !closed,
child: Padding(
padding: widget.childrenPadding ?? expansionTileTheme.childrenPadding ?? EdgeInsets.zero,
child: Column(
crossAxisAlignment: widget.expandedCrossAxisAlignment ?? CrossAxisAlignment.center,
children: widget.children,
),
),
),
);
return AnimatedBuilder(
animation: _animationController.view,
builder: _buildChildren,
child: shouldRemoveChildren ? null : result,
);
}
}
class _ExpansionTileDefaultsM2 extends ExpansionTileThemeData {
_ExpansionTileDefaultsM2(this.context);
final BuildContext context;
late final ThemeData _theme = Theme.of(context);
late final ColorScheme _colorScheme = _theme.colorScheme;
@override
Color? get textColor => _colorScheme.primary;
@override
Color? get iconColor => _colorScheme.primary;
@override
Color? get collapsedTextColor => _theme.textTheme.titleMedium!.color;
@override
Color? get collapsedIconColor => _theme.unselectedWidgetColor;
}
// BEGIN GENERATED TOKEN PROPERTIES - ExpansionTile
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _ExpansionTileDefaultsM3 extends ExpansionTileThemeData {
_ExpansionTileDefaultsM3(this.context);
final BuildContext context;
late final ThemeData _theme = Theme.of(context);
late final ColorScheme _colors = _theme.colorScheme;
@override
Color? get textColor => _colors.onSurface;
@override
Color? get iconColor => _colors.primary;
@override
Color? get collapsedTextColor => _colors.onSurface;
@override
Color? get collapsedIconColor => _colors.onSurfaceVariant;
}
// END GENERATED TOKEN PROPERTIES - ExpansionTile
| flutter/packages/flutter/lib/src/material/expansion_tile.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/expansion_tile.dart",
"repo_id": "flutter",
"token_count": 11182
} | 652 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'debug.dart';
import 'material.dart';
/// A convenience widget for drawing images and other decorations on [Material]
/// widgets, so that [InkWell] and [InkResponse] splashes will render over them.
///
/// Ink splashes and highlights, as rendered by [InkWell] and [InkResponse],
/// draw on the actual underlying [Material], under whatever widgets are drawn
/// over the material (such as [Text] and [Icon]s). If an opaque image is drawn
/// over the [Material] (maybe using a [Container] or [DecoratedBox]), these ink
/// effects will not be visible, as they will be entirely obscured by the opaque
/// graphics drawn above the [Material].
///
/// This widget draws the given [Decoration] directly on the [Material], in the
/// same way that [InkWell] and [InkResponse] draw there. This allows the
/// splashes to be drawn above the otherwise opaque graphics.
///
/// An alternative solution is to use a [MaterialType.transparency] material
/// above the opaque graphics, so that the ink responses from [InkWell]s and
/// [InkResponse]s will be drawn on the transparent material on top of the
/// opaque graphics, rather than under the opaque graphics on the underlying
/// [Material].
///
/// ## Limitations
///
/// This widget is subject to the same limitations as other ink effects, as
/// described in the documentation for [Material]. Most notably, the position of
/// an [Ink] widget must not change during the lifetime of the [Material] object
/// unless a [LayoutChangedNotification] is dispatched each frame that the
/// position changes. This is done automatically for [ListView] and other
/// scrolling widgets, but is not done for animated transitions such as
/// [SlideTransition].
///
/// Additionally, if multiple [Ink] widgets paint on the same [Material] in the
/// same location, their relative order is not guaranteed. The decorations will
/// be painted in the order that they were added to the material, which
/// generally speaking will match the order they are given in the widget tree,
/// but this order may appear to be somewhat random in more dynamic situations.
///
/// {@tool snippet}
///
/// This example shows how a [Material] widget can have a yellow rectangle drawn
/// on it using [Ink], while still having ink effects over the yellow rectangle:
///
/// ```dart
/// Material(
/// color: Colors.teal[900],
/// child: Center(
/// child: Ink(
/// color: Colors.yellow,
/// width: 200.0,
/// height: 100.0,
/// child: InkWell(
/// onTap: () { /* ... */ },
/// child: const Center(
/// child: Text('YELLOW'),
/// )
/// ),
/// ),
/// ),
/// )
/// ```
/// {@end-tool}
/// {@tool snippet}
///
/// The following example shows how an image can be printed on a [Material]
/// widget with an [InkWell] above it:
///
/// ```dart
/// Material(
/// color: Colors.grey[800],
/// child: Center(
/// child: Ink.image(
/// image: const AssetImage('cat.jpeg'),
/// fit: BoxFit.cover,
/// width: 300.0,
/// height: 200.0,
/// child: InkWell(
/// onTap: () { /* ... */ },
/// child: const Align(
/// alignment: Alignment.topLeft,
/// child: Padding(
/// padding: EdgeInsets.all(10.0),
/// child: Text(
/// 'KITTEN',
/// style: TextStyle(
/// fontWeight: FontWeight.w900,
/// color: Colors.white,
/// ),
/// ),
/// ),
/// )
/// ),
/// ),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// What to do if you want to clip this [Ink.image]?
///
/// {@tool dartpad}
/// Wrapping the [Ink] in a clipping widget directly will not work since the
/// [Material] it will be printed on is responsible for clipping.
///
/// In this example the image is not being clipped as expected. This is because
/// it is being rendered onto the Scaffold body Material, which isn't wrapped in
/// the [ClipRRect].
///
/// ** See code in examples/api/lib/material/ink/ink.image_clip.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// One solution would be to deliberately wrap the [Ink.image] in a [Material].
/// This makes sure the Material that the image is painted on is also responsible
/// for clipping said content.
///
/// ** See code in examples/api/lib/material/ink/ink.image_clip.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [Container], a more generic form of this widget which paints itself,
/// rather that deferring to the nearest [Material] widget.
/// * [InkDecoration], the [InkFeature] subclass used by this widget to paint
/// on [Material] widgets.
/// * [InkWell] and [InkResponse], which also draw on [Material] widgets.
class Ink extends StatefulWidget {
/// Paints a decoration (which can be a simple color) on a [Material].
///
/// The [height] and [width] values include the [padding].
///
/// The `color` argument is a shorthand for
/// `decoration: BoxDecoration(color: color)`, which means you cannot supply
/// both a `color` and a `decoration` argument. If you want to have both a
/// `color` and a `decoration`, you can pass the color as the `color`
/// argument to the `BoxDecoration`.
///
/// If there is no intention to render anything on this decoration, consider
/// using a [Container] with a [BoxDecoration] instead.
Ink({
super.key,
this.padding,
Color? color,
Decoration? decoration,
this.width,
this.height,
this.child,
}) : assert(padding == null || padding.isNonNegative),
assert(decoration == null || decoration.debugAssertIsValid()),
assert(color == null || decoration == null,
'Cannot provide both a color and a decoration\n'
'The color argument is just a shorthand for "decoration: BoxDecoration(color: color)".',
),
decoration = decoration ?? (color != null ? BoxDecoration(color: color) : null);
/// Creates a widget that shows an image (obtained from an [ImageProvider]) on
/// a [Material].
///
/// This argument is a shorthand for passing a [BoxDecoration] that has only
/// its [BoxDecoration.image] property set to the [Ink] constructor. The
/// properties of the [DecorationImage] of that [BoxDecoration] are set
/// according to the arguments passed to this method.
///
/// If there is no intention to render anything on this image, consider using
/// a [Container] with a [BoxDecoration.image] instead. The `onImageError`
/// argument may be provided to listen for errors when resolving the image.
///
/// The `alignment`, `repeat`, and `matchTextDirection` arguments must not
/// be null either, but they have default values.
///
/// See [paintImage] for a description of the meaning of these arguments.
Ink.image({
super.key,
this.padding,
required ImageProvider image,
ImageErrorListener? onImageError,
ColorFilter? colorFilter,
BoxFit? fit,
AlignmentGeometry alignment = Alignment.center,
Rect? centerSlice,
ImageRepeat repeat = ImageRepeat.noRepeat,
bool matchTextDirection = false,
this.width,
this.height,
this.child,
}) : assert(padding == null || padding.isNonNegative),
decoration = BoxDecoration(
image: DecorationImage(
image: image,
onError: onImageError,
colorFilter: colorFilter,
fit: fit,
alignment: alignment,
centerSlice: centerSlice,
repeat: repeat,
matchTextDirection: matchTextDirection,
),
);
/// The [child] contained by the container.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget? child;
/// Empty space to inscribe inside the [decoration]. The [child], if any, is
/// placed inside this padding.
///
/// This padding is in addition to any padding inherent in the [decoration];
/// see [Decoration.padding].
final EdgeInsetsGeometry? padding;
/// The decoration to paint on the nearest ancestor [Material] widget.
///
/// A shorthand for specifying just a solid color is available in the
/// constructor: set the `color` argument instead of the [decoration]
/// argument.
///
/// A shorthand for specifying just an image is also available using the
/// [Ink.image] constructor.
final Decoration? decoration;
/// A width to apply to the [decoration] and the [child]. The width includes
/// any [padding].
final double? width;
/// A height to apply to the [decoration] and the [child]. The height includes
/// any [padding].
final double? height;
EdgeInsetsGeometry get _paddingIncludingDecoration {
if (decoration == null) {
return padding ?? EdgeInsets.zero;
}
final EdgeInsetsGeometry decorationPadding = decoration!.padding;
if (padding == null) {
return decorationPadding;
}
return padding!.add(decorationPadding);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('padding', padding, defaultValue: null));
properties.add(DiagnosticsProperty<Decoration>('bg', decoration, defaultValue: null));
}
@override
State<Ink> createState() => _InkState();
}
class _InkState extends State<Ink> {
final GlobalKey _boxKey = GlobalKey();
InkDecoration? _ink;
void _handleRemoved() {
_ink = null;
}
@override
void deactivate() {
_ink?.dispose();
assert(_ink == null);
super.deactivate();
}
Widget _build(BuildContext context) {
// By creating the InkDecoration from within a Builder widget, we can
// use the RenderBox of the Padding widget.
if (_ink == null) {
_ink = InkDecoration(
decoration: widget.decoration,
isVisible: Visibility.of(context),
configuration: createLocalImageConfiguration(context),
controller: Material.of(context),
referenceBox: _boxKey.currentContext!.findRenderObject()! as RenderBox,
onRemoved: _handleRemoved,
);
} else {
_ink!.decoration = widget.decoration;
_ink!.isVisible = Visibility.of(context);
_ink!.configuration = createLocalImageConfiguration(context);
}
return widget.child ?? ConstrainedBox(constraints: const BoxConstraints.expand());
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterial(context));
Widget result = Padding(
key: _boxKey,
padding: widget._paddingIncludingDecoration,
child: Builder(builder: _build),
);
if (widget.width != null || widget.height != null) {
result = SizedBox(
width: widget.width,
height: widget.height,
child: result,
);
}
return result;
}
}
/// A decoration on a part of a [Material].
///
/// This object is rarely created directly. Instead of creating an ink
/// decoration directly, consider using an [Ink] widget, which uses this class
/// in combination with [Padding] and [ConstrainedBox] to draw a decoration on a
/// [Material].
///
/// See also:
///
/// * [Ink], the corresponding widget.
/// * [InkResponse], which uses gestures to trigger ink highlights and ink
/// splashes in the parent [Material].
/// * [InkWell], which is a rectangular [InkResponse] (the most common type of
/// ink response).
/// * [Material], which is the widget on which the ink is painted.
class InkDecoration extends InkFeature {
/// Draws a decoration on a [Material].
InkDecoration({
required Decoration? decoration,
bool isVisible = true,
required ImageConfiguration configuration,
required super.controller,
required super.referenceBox,
super.onRemoved,
}) : _configuration = configuration {
this.decoration = decoration;
this.isVisible = isVisible;
controller.addInkFeature(this);
}
BoxPainter? _painter;
/// What to paint on the [Material].
///
/// The decoration is painted at the position and size of the [referenceBox],
/// on the [Material] that owns the [controller].
Decoration? get decoration => _decoration;
Decoration? _decoration;
set decoration(Decoration? value) {
if (value == _decoration) {
return;
}
_decoration = value;
_painter?.dispose();
_painter = _decoration?.createBoxPainter(_handleChanged);
controller.markNeedsPaint();
}
/// Whether the decoration should be painted.
///
/// Defaults to true.
bool get isVisible => _isVisible;
bool _isVisible = true;
set isVisible(bool value) {
if (value == _isVisible) {
return;
}
_isVisible = value;
controller.markNeedsPaint();
}
/// The configuration to pass to the [BoxPainter] obtained from the
/// [decoration], when painting.
///
/// The [ImageConfiguration.size] field is ignored (and replaced by the size
/// of the [referenceBox], at paint time).
ImageConfiguration get configuration => _configuration;
ImageConfiguration _configuration;
set configuration(ImageConfiguration value) {
if (value == _configuration) {
return;
}
_configuration = value;
controller.markNeedsPaint();
}
void _handleChanged() {
controller.markNeedsPaint();
}
@override
void dispose() {
_painter?.dispose();
super.dispose();
}
@override
void paintFeature(Canvas canvas, Matrix4 transform) {
if (_painter == null || !isVisible) {
return;
}
final Offset? originOffset = MatrixUtils.getAsTranslation(transform);
final ImageConfiguration sizedConfiguration = configuration.copyWith(
size: referenceBox.size,
);
if (originOffset == null) {
canvas.save();
canvas.transform(transform.storage);
_painter!.paint(canvas, Offset.zero, sizedConfiguration);
canvas.restore();
} else {
_painter!.paint(canvas, originOffset, sizedConfiguration);
}
}
}
| flutter/packages/flutter/lib/src/material/ink_decoration.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/ink_decoration.dart",
"repo_id": "flutter",
"token_count": 4634
} | 653 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'input_border.dart';
// Examples can assume:
// late BuildContext context;
/// Interactive states that some of the Material widgets can take on when
/// receiving input from the user.
///
/// States are defined by https://material.io/design/interaction/states.html#usage.
///
/// Some Material widgets track their current state in a `Set<MaterialState>`.
///
/// See also:
///
/// * [WidgetState], a general non-Material version that can be used
/// interchangebly with `MaterialState`. They functionally work the same,
/// except [WidgetState] can be used outside of Material.
/// * [MaterialStateProperty], an interface for objects that "resolve" to
/// different values depending on a widget's material state.
/// {@template flutter.material.MaterialStateProperty.implementations}
/// * [MaterialStateColor], a [Color] that implements `MaterialStateProperty`
/// which is used in APIs that need to accept either a [Color] or a
/// `MaterialStateProperty<Color>`.
/// * [MaterialStateMouseCursor], a [MouseCursor] that implements
/// `MaterialStateProperty` which is used in APIs that need to accept either
/// a [MouseCursor] or a [MaterialStateProperty<MouseCursor>].
/// * [MaterialStateOutlinedBorder], an [OutlinedBorder] that implements
/// `MaterialStateProperty` which is used in APIs that need to accept either
/// an [OutlinedBorder] or a [MaterialStateProperty<OutlinedBorder>].
/// * [MaterialStateOutlineInputBorder], an [OutlineInputBorder] that implements
/// `MaterialStateProperty` which is used in APIs that need to accept either
/// an [OutlineInputBorder] or a [MaterialStateProperty<OutlineInputBorder>].
/// * [MaterialStateUnderlineInputBorder], an [UnderlineInputBorder] that implements
/// `MaterialStateProperty` which is used in APIs that need to accept either
/// an [UnderlineInputBorder] or a [MaterialStateProperty<UnderlineInputBorder>].
/// * [MaterialStateBorderSide], a [BorderSide] that implements
/// `MaterialStateProperty` which is used in APIs that need to accept either
/// a [BorderSide] or a [MaterialStateProperty<BorderSide>].
/// * [MaterialStateTextStyle], a [TextStyle] that implements
/// `MaterialStateProperty` which is used in APIs that need to accept either
/// a [TextStyle] or a [MaterialStateProperty<TextStyle>].
/// {@endtemplate}
@Deprecated(
'Use WidgetState instead. '
'Moved to the Widgets layer to make code available outside of Material. '
'This feature was deprecated after v3.19.0-0.3.pre.'
)
typedef MaterialState = WidgetState;
/// Signature for the function that returns a value of type `T` based on a given
/// set of states.
///
/// See also:
///
/// * [WidgetPropertyResolver], the non-Material form of `MaterialPropertyResolver`
/// that can be used interchangably with `MaterialPropertyResolver.
@Deprecated(
'Use WidgetPropertyResolver instead. '
'Moved to the Widgets layer to make code available outside of Material. '
'This feature was deprecated after v3.19.0-0.3.pre.'
)
typedef MaterialPropertyResolver<T> = WidgetPropertyResolver<T>;
/// Defines a [Color] that is also a [MaterialStateProperty].
///
/// This class exists to enable widgets with [Color] valued properties
/// to also accept [MaterialStateProperty<Color>] values. A material
/// state color property represents a color which depends on
/// a widget's "interactive state". This state is represented as a
/// [Set] of [MaterialState]s, like [MaterialState.pressed],
/// [MaterialState.focused] and [MaterialState.hovered].
///
/// [MaterialStateColor] should only be used with widgets that document
/// their support, like [TimePickerThemeData.dayPeriodColor].
///
/// To use a [MaterialStateColor], you can either:
/// 1. Create a subclass of [MaterialStateColor] and implement the abstract `resolve` method.
/// 2. Use [MaterialStateColor.resolveWith] and pass in a callback that
/// will be used to resolve the color in the given states.
///
/// If a [MaterialStateColor] is used for a property or a parameter that doesn't
/// support resolving [MaterialStateProperty<Color>]s, then its default color
/// value will be used for all states.
///
/// To define a `const` [MaterialStateColor], you'll need to extend
/// [MaterialStateColor] and override its [resolve] method. You'll also need
/// to provide a `defaultValue` to the super constructor, so that we can know
/// at compile-time what its default color is.
///
/// {@tool snippet}
///
/// This example defines a [MaterialStateColor] with a const constructor.
///
/// ```dart
/// class MyColor extends MaterialStateColor {
/// const MyColor() : super(_defaultColor);
///
/// static const int _defaultColor = 0xcafefeed;
/// static const int _pressedColor = 0xdeadbeef;
///
/// @override
/// Color resolve(Set<MaterialState> states) {
/// if (states.contains(MaterialState.pressed)) {
/// return const Color(_pressedColor);
/// }
/// return const Color(_defaultColor);
/// }
/// }
/// ```
/// {@end-tool}
///
/// See also
///
/// * [WidgetStateColor], the non-Material version that can be used
/// interchangably with `MaterialStateColor`.
@Deprecated(
'Use WidgetStateColor instead. '
'Moved to the Widgets layer to make code available outside of Material. '
'This feature was deprecated after v3.19.0-0.3.pre.'
)
typedef MaterialStateColor = WidgetStateColor;
/// Defines a [MouseCursor] whose value depends on a set of [MaterialState]s which
/// represent the interactive state of a component.
///
/// This kind of [MouseCursor] is useful when the set of interactive
/// actions a widget supports varies with its state. For example, a
/// mouse pointer hovering over a disabled [ListTile] should not
/// display [SystemMouseCursors.click], since a disabled list tile
/// doesn't respond to mouse clicks. [ListTile]'s default mouse cursor
/// is a [MaterialStateMouseCursor.clickable], which resolves to
/// [SystemMouseCursors.basic] when the button is disabled.
///
/// To use a [MaterialStateMouseCursor], you should create a subclass of
/// [MaterialStateMouseCursor] and implement the abstract `resolve` method.
///
/// {@tool dartpad}
/// This example defines a mouse cursor that resolves to
/// [SystemMouseCursors.forbidden] when its widget is disabled.
///
/// ** See code in examples/api/lib/material/material_state/material_state_mouse_cursor.0.dart **
/// {@end-tool}
///
/// This class should only be used for parameters which are documented to take
/// [MaterialStateMouseCursor], otherwise only the default state will be used.
///
/// See also:
///
/// * [WidgetStateMouseCursor], the non-Material version that can be used
/// interchangeably with `MaterialStateMouseCursor`.
/// * [MouseCursor] for introduction on the mouse cursor system.
/// * [SystemMouseCursors], which defines cursors that are supported by
/// native platforms.
@Deprecated(
'Use WidgetStateMouseCursor instead. '
'Moved to the Widgets layer to make code available outside of Material. '
'This feature was deprecated after v3.19.0-0.3.pre.'
)
typedef MaterialStateMouseCursor = WidgetStateMouseCursor;
/// Defines a [BorderSide] whose value depends on a set of [MaterialState]s
/// which represent the interactive state of a component.
///
/// To use a [MaterialStateBorderSide], you should create a subclass of a
/// [MaterialStateBorderSide] and override the abstract `resolve` method.
///
/// This class enables existing widget implementations with [BorderSide]
/// properties to be extended to also effectively support `MaterialStateProperty<BorderSide>`
/// property values. [MaterialStateBorderSide] should only be used with widgets that document
/// their support, like [ActionChip.side].
///
/// {@tool dartpad}
/// This example defines a subclass of [MaterialStateBorderSide], that resolves
/// to a red border side when its widget is selected.
///
/// ** See code in examples/api/lib/material/material_state/material_state_border_side.0.dart **
/// {@end-tool}
///
/// This class should only be used for parameters which are documented to take
/// [MaterialStateBorderSide], otherwise only the default state will be used.
///
/// See also:
///
/// * [WidgetStateBorderSide], the non-Material version that can be used
/// interchangeably with `MaterialStateBorderSide`.
@Deprecated(
'Use WidgetStateBorderSide instead. '
'Moved to the Widgets layer to make code available outside of Material. '
'This feature was deprecated after v3.19.0-0.3.pre.'
)
typedef MaterialStateBorderSide = WidgetStateBorderSide;
/// Defines an [OutlinedBorder] whose value depends on a set of [MaterialState]s
/// which represent the interactive state of a component.
///
/// To use a [MaterialStateOutlinedBorder], you should create a subclass of an
/// [OutlinedBorder] and implement [MaterialStateOutlinedBorder]'s abstract
/// `resolve` method.
///
/// {@tool dartpad}
/// This example defines a subclass of [RoundedRectangleBorder] and an
/// implementation of [MaterialStateOutlinedBorder], that resolves to
/// [RoundedRectangleBorder] when its widget is selected.
///
/// ** See code in examples/api/lib/material/material_state/material_state_outlined_border.0.dart **
/// {@end-tool}
///
/// This class should only be used for parameters which are documented to take
/// [MaterialStateOutlinedBorder], otherwise only the default state will be used.
///
/// See also:
///
/// * [WidgetStateOutlinedBorder], the non-Material version that can be used
/// interchangeably with `MaterialStateOutlinedBorder`.
/// * [ShapeBorder] the base class for shape outlines.
@Deprecated(
'Use WidgetStateOutlinedBorder instead. '
'Moved to the Widgets layer to make code available outside of Material. '
'This feature was deprecated after v3.19.0-0.3.pre.'
)
typedef MaterialStateOutlinedBorder = WidgetStateOutlinedBorder;
/// Defines a [TextStyle] that is also a [MaterialStateProperty].
///
/// This class exists to enable widgets with [TextStyle] valued properties
/// to also accept [MaterialStateProperty<TextStyle>] values. A material
/// state text style property represents a text style which depends on
/// a widget's "interactive state". This state is represented as a
/// [Set] of [MaterialState]s, like [MaterialState.pressed],
/// [MaterialState.focused] and [MaterialState.hovered].
///
/// [MaterialStateTextStyle] should only be used with widgets that document
/// their support, like [InputDecoration.labelStyle].
///
/// To use a [MaterialStateTextStyle], you can either:
/// 1. Create a subclass of [MaterialStateTextStyle] and implement the abstract `resolve` method.
/// 2. Use [MaterialStateTextStyle.resolveWith] and pass in a callback that
/// will be used to resolve the color in the given states.
///
/// If a [MaterialStateTextStyle] is used for a property or a parameter that doesn't
/// support resolving [MaterialStateProperty<TextStyle>]s, then its default color
/// value will be used for all states.
///
/// To define a `const` [MaterialStateTextStyle], you'll need to extend
/// [MaterialStateTextStyle] and override its [resolve] method. You'll also need
/// to provide a `defaultValue` to the super constructor, so that we can know
/// at compile-time what its default color is.
///
/// See also:
///
/// * [WidgetStateTextStyle], the non-Material version that can be used
/// interchangeably with `MaterialStateTextStyle`.
@Deprecated(
'Use WidgetStateTextStyle instead. '
'Moved to the Widgets layer to make code available outside of Material. '
'This feature was deprecated after v3.19.0-0.3.pre.'
)
typedef MaterialStateTextStyle = WidgetStateTextStyle;
/// Defines a [OutlineInputBorder] that is also a [MaterialStateProperty].
///
/// This class exists to enable widgets with [OutlineInputBorder] valued properties
/// to also accept [MaterialStateProperty<OutlineInputBorder>] values. A material
/// state input border property represents a text style which depends on
/// a widget's "interactive state". This state is represented as a
/// [Set] of [MaterialState]s, like [MaterialState.pressed],
/// [MaterialState.focused] and [MaterialState.hovered].
///
/// [MaterialStateOutlineInputBorder] should only be used with widgets that document
/// their support, like [InputDecoration.border].
///
/// To use a [MaterialStateOutlineInputBorder], you can either:
/// 1. Create a subclass of [MaterialStateOutlineInputBorder] and implement the abstract `resolve` method.
/// 2. Use [MaterialStateOutlineInputBorder.resolveWith] and pass in a callback that
/// will be used to resolve the color in the given states.
///
/// If a [MaterialStateOutlineInputBorder] is used for a property or a parameter that doesn't
/// support resolving [MaterialStateProperty<OutlineInputBorder>]s, then its default color
/// value will be used for all states.
///
/// To define a `const` [MaterialStateOutlineInputBorder], you'll need to extend
/// [MaterialStateOutlineInputBorder] and override its [resolve] method. You'll also need
/// to provide a `defaultValue` to the super constructor, so that we can know
/// at compile-time what its default color is.
abstract class MaterialStateOutlineInputBorder extends OutlineInputBorder implements MaterialStateProperty<InputBorder> {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const MaterialStateOutlineInputBorder();
/// Creates a [MaterialStateOutlineInputBorder] from a [MaterialPropertyResolver<InputBorder>]
/// callback function.
///
/// If used as a regular input border, the border resolved in the default state (the
/// empty set of states) will be used.
///
/// The given callback parameter must return a non-null text style in the default
/// state.
const factory MaterialStateOutlineInputBorder.resolveWith(MaterialPropertyResolver<InputBorder> callback) = _MaterialStateOutlineInputBorder;
/// Returns a [InputBorder] that's to be used when a Material component is in the
/// specified state.
@override
InputBorder resolve(Set<MaterialState> states);
}
/// A [MaterialStateOutlineInputBorder] created from a [MaterialPropertyResolver<OutlineInputBorder>]
/// callback alone.
///
/// If used as a regular input border, the border resolved in the default state will
/// be used.
///
/// Used by [MaterialStateTextStyle.resolveWith].
class _MaterialStateOutlineInputBorder extends MaterialStateOutlineInputBorder {
const _MaterialStateOutlineInputBorder(this._resolve);
final MaterialPropertyResolver<InputBorder> _resolve;
@override
InputBorder resolve(Set<MaterialState> states) => _resolve(states);
}
/// Defines a [UnderlineInputBorder] that is also a [MaterialStateProperty].
///
/// This class exists to enable widgets with [UnderlineInputBorder] valued properties
/// to also accept [MaterialStateProperty<UnderlineInputBorder>] values. A material
/// state input border property represents a text style which depends on
/// a widget's "interactive state". This state is represented as a
/// [Set] of [MaterialState]s, like [MaterialState.pressed],
/// [MaterialState.focused] and [MaterialState.hovered].
///
/// [MaterialStateUnderlineInputBorder] should only be used with widgets that document
/// their support, like [InputDecoration.border].
///
/// To use a [MaterialStateUnderlineInputBorder], you can either:
/// 1. Create a subclass of [MaterialStateUnderlineInputBorder] and implement the abstract `resolve` method.
/// 2. Use [MaterialStateUnderlineInputBorder.resolveWith] and pass in a callback that
/// will be used to resolve the color in the given states.
///
/// If a [MaterialStateUnderlineInputBorder] is used for a property or a parameter that doesn't
/// support resolving [MaterialStateProperty<UnderlineInputBorder>]s, then its default color
/// value will be used for all states.
///
/// To define a `const` [MaterialStateUnderlineInputBorder], you'll need to extend
/// [MaterialStateUnderlineInputBorder] and override its [resolve] method. You'll also need
/// to provide a `defaultValue` to the super constructor, so that we can know
/// at compile-time what its default color is.
abstract class MaterialStateUnderlineInputBorder extends UnderlineInputBorder implements MaterialStateProperty<InputBorder> {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const MaterialStateUnderlineInputBorder();
/// Creates a [MaterialStateUnderlineInputBorder] from a [MaterialPropertyResolver<InputBorder>]
/// callback function.
///
/// If used as a regular input border, the border resolved in the default state (the
/// empty set of states) will be used.
///
/// The given callback parameter must return a non-null text style in the default
/// state.
const factory MaterialStateUnderlineInputBorder.resolveWith(MaterialPropertyResolver<InputBorder> callback) = _MaterialStateUnderlineInputBorder;
/// Returns a [InputBorder] that's to be used when a Material component is in the
/// specified state.
@override
InputBorder resolve(Set<MaterialState> states);
}
/// A [MaterialStateUnderlineInputBorder] created from a [MaterialPropertyResolver<UnderlineInputBorder>]
/// callback alone.
///
/// If used as a regular input border, the border resolved in the default state will
/// be used.
///
/// Used by [MaterialStateTextStyle.resolveWith].
class _MaterialStateUnderlineInputBorder extends MaterialStateUnderlineInputBorder {
const _MaterialStateUnderlineInputBorder(this._resolve);
final MaterialPropertyResolver<InputBorder> _resolve;
@override
InputBorder resolve(Set<MaterialState> states) => _resolve(states);
}
/// Interface for classes that [resolve] to a value of type `T` based
/// on a widget's interactive "state", which is defined as a set
/// of [MaterialState]s.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=CylXr3AF3uU}
///
/// Material state properties represent values that depend on a widget's material
/// "state". The state is encoded as a set of [MaterialState] values, like
/// [MaterialState.focused], [MaterialState.hovered], [MaterialState.pressed]. For
/// example the [InkWell.overlayColor] defines the color that fills the ink well
/// when it's pressed (the "splash color"), focused, or hovered. The [InkWell]
/// uses the overlay color's [resolve] method to compute the color for the
/// ink well's current state.
///
/// [ButtonStyle], which is used to configure the appearance of
/// buttons like [TextButton], [ElevatedButton], and [OutlinedButton],
/// has many material state properties. The button widgets keep track
/// of their current material state and [resolve] the button style's
/// material state properties when their value is needed.
///
/// {@tool dartpad}
/// This example shows how you can override the default text and icon
/// color (the "foreground color") of a [TextButton] with a
/// [MaterialStateProperty]. In this example, the button's text color
/// will be `Colors.blue` when the button is being pressed, hovered,
/// or focused. Otherwise, the text color will be `Colors.red`.
///
/// ** See code in examples/api/lib/material/material_state/material_state_property.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [WidgetStateProperty], the non-Material version that can be used
/// interchangeably with `MaterialStateProperty`.
/// {@macro flutter.material.MaterialStateProperty.implementations}
@Deprecated(
'Use WidgetStateProperty instead. '
'Moved to the Widgets layer to make code available outside of Material. '
'This feature was deprecated after v3.19.0-0.3.pre.'
)
typedef MaterialStateProperty<T> = WidgetStateProperty<T>;
/// Convenience class for creating a [MaterialStateProperty] that
/// resolves to the given value for all states.
///
/// See also:
///
/// * [WidgetStatePropertyAll], the non-Material version that can be used
/// interchangeably with `MaterialStatePropertyAll`.
@Deprecated(
'Use WidgetStatePropertyAll instead. '
'Moved to the Widgets layer to make code available outside of Material. '
'This feature was deprecated after v3.19.0-0.3.pre.'
)
typedef MaterialStatePropertyAll<T> = WidgetStatePropertyAll<T>;
/// Manages a set of [MaterialState]s and notifies listeners of changes.
///
/// Used by widgets that expose their internal state for the sake of
/// extensions that add support for additional states. See
/// [TextButton] for an example.
///
/// The controller's [value] is its current set of states. Listeners
/// are notified whenever the [value] changes. The [value] should only be
/// changed with [update]; it should not be modified directly.
///
/// The controller's [value] represents the set of states that a
/// widget's visual properties, typically [MaterialStateProperty]
/// values, are resolved against. It is _not_ the intrinsic state of
/// the widget. The widget is responsible for ensuring that the
/// controller's [value] tracks its intrinsic state. For example one
/// cannot request the keyboard focus for a widget by adding
/// [MaterialState.focused] to its controller. When the widget gains the
/// or loses the focus it will [update] its controller's [value] and
/// notify listeners of the change.
///
/// When calling `setState` in a [MaterialStatesController] listener, use the
/// [SchedulerBinding.addPostFrameCallback] to delay the call to `setState` after
/// the frame has been rendered. It's generally prudent to use the
/// [SchedulerBinding.addPostFrameCallback] because some of the widgets that
/// depend on [MaterialStatesController] may call [update] in their build method.
/// In such cases, listener's that call `setState` - during the build phase - will cause
/// an error.
///
/// See also:
///
/// * [WidgetStatesController], the non-Material version that can be used
/// interchangeably with `MaterialStatesController`.
@Deprecated(
'Use WidgetStatesController instead. '
'Moved to the Widgets layer to make code available outside of Material. '
'This feature was deprecated after v3.19.0-0.3.pre.'
)
typedef MaterialStatesController = WidgetStatesController;
| flutter/packages/flutter/lib/src/material/material_state.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/material_state.dart",
"repo_id": "flutter",
"token_count": 5938
} | 654 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/widgets.dart';
import 'button_style.dart';
import 'button_style_button.dart';
import 'color_scheme.dart';
import 'colors.dart';
import 'constants.dart';
import 'ink_ripple.dart';
import 'ink_well.dart';
import 'material_state.dart';
import 'outlined_button_theme.dart';
import 'theme.dart';
import 'theme_data.dart';
/// A Material Design "Outlined Button"; essentially a [TextButton]
/// with an outlined border.
///
/// Outlined buttons are medium-emphasis buttons. They contain actions
/// that are important, but they aren’t the primary action in an app.
///
/// An outlined button is a label [child] displayed on a (zero
/// elevation) [Material] widget. The label's [Text] and [Icon]
/// widgets are displayed in the [style]'s
/// [ButtonStyle.foregroundColor] and the outline's weight and color
/// are defined by [ButtonStyle.side]. The button reacts to touches
/// by filling with the [style]'s [ButtonStyle.overlayColor].
///
/// The outlined button's default style is defined by [defaultStyleOf].
/// The style of this outline button can be overridden with its [style]
/// parameter. The style of all text buttons in a subtree can be
/// overridden with the [OutlinedButtonTheme] and the style of all of the
/// outlined buttons in an app can be overridden with the [Theme]'s
/// [ThemeData.outlinedButtonTheme] property.
///
/// Unlike [TextButton] or [ElevatedButton], outline buttons have a
/// default [ButtonStyle.side] which defines the appearance of the
/// outline. Because the default `side` is non-null, it
/// unconditionally overrides the shape's [OutlinedBorder.side]. In
/// other words, to specify an outlined button's shape _and_ the
/// appearance of its outline, both the [ButtonStyle.shape] and
/// [ButtonStyle.side] properties must be specified.
///
/// {@tool dartpad}
/// Here is an example of a basic [OutlinedButton].
///
/// ** See code in examples/api/lib/material/outlined_button/outlined_button.0.dart **
/// {@end-tool}
///
/// The static [styleFrom] method is a convenient way to create a
/// outlined button [ButtonStyle] from simple values.
///
/// See also:
///
/// * [ElevatedButton], a filled button whose material elevates when pressed.
/// * [FilledButton], a filled button that doesn't elevate when pressed.
/// * [FilledButton.tonal], a filled button variant that uses a secondary fill color.
/// * [TextButton], a button with no outline or fill color.
/// * <https://material.io/design/components/buttons.html>
/// * <https://m3.material.io/components/buttons>
class OutlinedButton extends ButtonStyleButton {
/// Create an OutlinedButton.
const OutlinedButton({
super.key,
required super.onPressed,
super.onLongPress,
super.onHover,
super.onFocusChange,
super.style,
super.focusNode,
super.autofocus = false,
super.clipBehavior,
super.statesController,
required super.child,
super.iconAlignment,
});
/// Create a text button from a pair of widgets that serve as the button's
/// [icon] and [label].
///
/// The icon and label are arranged in a row and padded by 12 logical pixels
/// at the start, and 16 at the end, with an 8 pixel gap in between.
///
/// If [icon] is null, will create an [OutlinedButton] instead.
///
/// {@macro flutter.material.ButtonStyleButton.iconAlignment}
///
factory OutlinedButton.icon({
Key? key,
required VoidCallback? onPressed,
VoidCallback? onLongPress,
ButtonStyle? style,
FocusNode? focusNode,
bool? autofocus,
Clip? clipBehavior,
MaterialStatesController? statesController,
Widget? icon,
required Widget label,
IconAlignment iconAlignment = IconAlignment.start,
}) {
if (icon == null) {
return OutlinedButton(
key: key,
onPressed: onPressed,
onLongPress: onLongPress,
style: style,
focusNode: focusNode,
autofocus: autofocus ?? false,
clipBehavior: clipBehavior ?? Clip.none,
statesController: statesController,
child: label,
);
}
return _OutlinedButtonWithIcon(
key: key,
onPressed: onPressed,
onLongPress: onLongPress,
style: style,
focusNode: focusNode,
autofocus: autofocus ?? false,
clipBehavior: clipBehavior ?? Clip.none,
statesController: statesController,
icon: icon,
label: label,
iconAlignment: iconAlignment,
);
}
/// A static convenience method that constructs an outlined button
/// [ButtonStyle] given simple values.
///
/// The [foregroundColor] and [disabledForegroundColor] colors are used
/// to create a [MaterialStateProperty] [ButtonStyle.foregroundColor], and
/// a derived [ButtonStyle.overlayColor] if [overlayColor] isn't specified.
///
/// The [backgroundColor] and [disabledBackgroundColor] colors are
/// used to create a [MaterialStateProperty] [ButtonStyle.backgroundColor].
///
/// Similarly, the [enabledMouseCursor] and [disabledMouseCursor]
/// parameters are used to construct [ButtonStyle.mouseCursor] and
/// [iconColor], [disabledIconColor] are used to construct
/// [ButtonStyle.iconColor].
///
/// If [overlayColor] is specified and its value is [Colors.transparent]
/// then the pressed/focused/hovered highlights are effectively defeated.
/// Otherwise a [MaterialStateProperty] with the same opacities as the
/// default is created.
///
/// All of the other parameters are either used directly or used to
/// create a [MaterialStateProperty] with a single value for all
/// states.
///
/// All parameters default to null, by default this method returns
/// a [ButtonStyle] that doesn't override anything.
///
/// For example, to override the default shape and outline for an
/// [OutlinedButton], one could write:
///
/// ```dart
/// OutlinedButton(
/// style: OutlinedButton.styleFrom(
/// shape: const StadiumBorder(),
/// side: const BorderSide(width: 2, color: Colors.green),
/// ),
/// child: const Text('Seasons of Love'),
/// onPressed: () {
/// // ...
/// },
/// ),
/// ```
static ButtonStyle styleFrom({
Color? foregroundColor,
Color? backgroundColor,
Color? disabledForegroundColor,
Color? disabledBackgroundColor,
Color? shadowColor,
Color? surfaceTintColor,
Color? iconColor,
Color? disabledIconColor,
Color? overlayColor,
double? elevation,
TextStyle? textStyle,
EdgeInsetsGeometry? padding,
Size? minimumSize,
Size? fixedSize,
Size? maximumSize,
BorderSide? side,
OutlinedBorder? shape,
MouseCursor? enabledMouseCursor,
MouseCursor? disabledMouseCursor,
VisualDensity? visualDensity,
MaterialTapTargetSize? tapTargetSize,
Duration? animationDuration,
bool? enableFeedback,
AlignmentGeometry? alignment,
InteractiveInkFeatureFactory? splashFactory,
ButtonLayerBuilder? backgroundBuilder,
ButtonLayerBuilder? foregroundBuilder,
}) {
final MaterialStateProperty<Color?>? foregroundColorProp = switch ((foregroundColor, disabledForegroundColor)) {
(null, null) => null,
(_, _) => _OutlinedButtonDefaultColor(foregroundColor, disabledForegroundColor),
};
final MaterialStateProperty<Color?>? backgroundColorProp = switch ((backgroundColor, disabledBackgroundColor)) {
(null, null) => null,
(_, null) => MaterialStatePropertyAll<Color?>(backgroundColor),
(_, _) => _OutlinedButtonDefaultColor(backgroundColor, disabledBackgroundColor),
};
final MaterialStateProperty<Color?>? iconColorProp = switch ((iconColor, disabledIconColor)) {
(null, null) => null,
(_, _) => _OutlinedButtonDefaultColor(iconColor, disabledIconColor),
};
final MaterialStateProperty<Color?>? overlayColorProp = switch ((foregroundColor, overlayColor)) {
(null, null) => null,
(_, final Color overlayColor) when overlayColor.value == 0 => const MaterialStatePropertyAll<Color?>(Colors.transparent),
(_, _) => _OutlinedButtonDefaultOverlay((overlayColor ?? foregroundColor)!),
};
final MaterialStateProperty<MouseCursor?> mouseCursor = _OutlinedButtonDefaultMouseCursor(enabledMouseCursor, disabledMouseCursor);
return ButtonStyle(
textStyle: ButtonStyleButton.allOrNull<TextStyle>(textStyle),
foregroundColor: foregroundColorProp,
backgroundColor: backgroundColorProp,
overlayColor: overlayColorProp,
shadowColor: ButtonStyleButton.allOrNull<Color>(shadowColor),
surfaceTintColor: ButtonStyleButton.allOrNull<Color>(surfaceTintColor),
iconColor: iconColorProp,
elevation: ButtonStyleButton.allOrNull<double>(elevation),
padding: ButtonStyleButton.allOrNull<EdgeInsetsGeometry>(padding),
minimumSize: ButtonStyleButton.allOrNull<Size>(minimumSize),
fixedSize: ButtonStyleButton.allOrNull<Size>(fixedSize),
maximumSize: ButtonStyleButton.allOrNull<Size>(maximumSize),
side: ButtonStyleButton.allOrNull<BorderSide>(side),
shape: ButtonStyleButton.allOrNull<OutlinedBorder>(shape),
mouseCursor: mouseCursor,
visualDensity: visualDensity,
tapTargetSize: tapTargetSize,
animationDuration: animationDuration,
enableFeedback: enableFeedback,
alignment: alignment,
splashFactory: splashFactory,
backgroundBuilder: backgroundBuilder,
foregroundBuilder: foregroundBuilder,
);
}
/// Defines the button's default appearance.
///
/// With the exception of [ButtonStyle.side], which defines the
/// outline, and [ButtonStyle.padding], the returned style is the
/// same as for [TextButton].
///
/// The button [child]'s [Text] and [Icon] widgets are rendered with
/// the [ButtonStyle]'s foreground color. The button's [InkWell] adds
/// the style's overlay color when the button is focused, hovered
/// or pressed. The button's background color becomes its [Material]
/// color and is transparent by default.
///
/// All of the ButtonStyle's defaults appear below. In this list
/// "Theme.foo" is shorthand for `Theme.of(context).foo`. Color
/// scheme values like "onSurface(0.38)" are shorthand for
/// `onSurface.withOpacity(0.38)`. [MaterialStateProperty] valued
/// properties that are not followed by a sublist have the same
/// value for all states, otherwise the values are as specified for
/// each state and "others" means all other states.
///
/// The color of the [ButtonStyle.textStyle] is not used, the
/// [ButtonStyle.foregroundColor] is used instead.
///
/// ## Material 2 defaults
///
/// * `textStyle` - Theme.textTheme.button
/// * `backgroundColor` - transparent
/// * `foregroundColor`
/// * disabled - Theme.colorScheme.onSurface(0.38)
/// * others - Theme.colorScheme.primary
/// * `overlayColor`
/// * hovered - Theme.colorScheme.primary(0.08)
/// * focused or pressed - Theme.colorScheme.primary(0.12)
/// * `shadowColor` - Theme.shadowColor
/// * `elevation` - 0
/// * `padding`
/// * `default font size <= 14` - horizontal(16)
/// * `14 < default font size <= 28` - lerp(horizontal(16), horizontal(8))
/// * `28 < default font size <= 36` - lerp(horizontal(8), horizontal(4))
/// * `36 < default font size` - horizontal(4)
/// * `minimumSize` - Size(64, 36)
/// * `fixedSize` - null
/// * `maximumSize` - Size.infinite
/// * `side` - BorderSide(width: 1, color: Theme.colorScheme.onSurface(0.12))
/// * `shape` - RoundedRectangleBorder(borderRadius: BorderRadius.circular(4))
/// * `mouseCursor`
/// * disabled - SystemMouseCursors.basic
/// * others - SystemMouseCursors.click
/// * `visualDensity` - theme.visualDensity
/// * `tapTargetSize` - theme.materialTapTargetSize
/// * `animationDuration` - kThemeChangeDuration
/// * `enableFeedback` - true
/// * `alignment` - Alignment.center
/// * `splashFactory` - InkRipple.splashFactory
///
/// ## Material 3 defaults
///
/// If [ThemeData.useMaterial3] is set to true the following defaults will
/// be used:
///
/// * `textStyle` - Theme.textTheme.labelLarge
/// * `backgroundColor` - transparent
/// * `foregroundColor`
/// * disabled - Theme.colorScheme.onSurface(0.38)
/// * others - Theme.colorScheme.primary
/// * `overlayColor`
/// * hovered - Theme.colorScheme.primary(0.08)
/// * focused or pressed - Theme.colorScheme.primary(0.1)
/// * others - null
/// * `shadowColor` - Colors.transparent,
/// * `surfaceTintColor` - null
/// * `elevation` - 0
/// * `padding`
/// * `default font size <= 14` - horizontal(24)
/// * `14 < default font size <= 28` - lerp(horizontal(24), horizontal(12))
/// * `28 < default font size <= 36` - lerp(horizontal(12), horizontal(6))
/// * `36 < default font size` - horizontal(6)
/// * `minimumSize` - Size(64, 40)
/// * `fixedSize` - null
/// * `maximumSize` - Size.infinite
/// * `side`
/// * disabled - BorderSide(color: Theme.colorScheme.onSurface(0.12))
/// * others - BorderSide(color: Theme.colorScheme.outline)
/// * `shape` - StadiumBorder()
/// * `mouseCursor`
/// * disabled - SystemMouseCursors.basic
/// * others - SystemMouseCursors.click
/// * `visualDensity` - theme.visualDensity
/// * `tapTargetSize` - theme.materialTapTargetSize
/// * `animationDuration` - kThemeChangeDuration
/// * `enableFeedback` - true
/// * `alignment` - Alignment.center
/// * `splashFactory` - Theme.splashFactory
///
/// For the [OutlinedButton.icon] factory, the start (generally the left) value of
/// [padding] is reduced from 24 to 16.
@override
ButtonStyle defaultStyleOf(BuildContext context) {
final ThemeData theme = Theme.of(context);
final ColorScheme colorScheme = theme.colorScheme;
return Theme.of(context).useMaterial3
? _OutlinedButtonDefaultsM3(context)
: styleFrom(
foregroundColor: colorScheme.primary,
disabledForegroundColor: colorScheme.onSurface.withOpacity(0.38),
backgroundColor: Colors.transparent,
disabledBackgroundColor: Colors.transparent,
shadowColor: theme.shadowColor,
elevation: 0,
textStyle: theme.textTheme.labelLarge,
padding: _scaledPadding(context),
minimumSize: const Size(64, 36),
maximumSize: Size.infinite,
side: BorderSide(color: colorScheme.onSurface.withOpacity(0.12)),
shape: const RoundedRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(4))),
enabledMouseCursor: SystemMouseCursors.click,
disabledMouseCursor: SystemMouseCursors.basic,
visualDensity: theme.visualDensity,
tapTargetSize: theme.materialTapTargetSize,
animationDuration: kThemeChangeDuration,
enableFeedback: true,
alignment: Alignment.center,
splashFactory: InkRipple.splashFactory,
);
}
@override
ButtonStyle? themeStyleOf(BuildContext context) {
return OutlinedButtonTheme.of(context).style;
}
}
EdgeInsetsGeometry _scaledPadding(BuildContext context) {
final ThemeData theme = Theme.of(context);
final double padding1x = theme.useMaterial3 ? 24.0 : 16.0;
final double defaultFontSize = theme.textTheme.labelLarge?.fontSize ?? 14.0;
final double effectiveTextScale = MediaQuery.textScalerOf(context).scale(defaultFontSize) / 14.0;
return ButtonStyleButton.scaledPadding(
EdgeInsets.symmetric(horizontal: padding1x),
EdgeInsets.symmetric(horizontal: padding1x / 2),
EdgeInsets.symmetric(horizontal: padding1x / 2 / 2),
effectiveTextScale,
);
}
@immutable
class _OutlinedButtonDefaultColor extends MaterialStateProperty<Color?> with Diagnosticable {
_OutlinedButtonDefaultColor(this.color, this.disabled);
final Color? color;
final Color? disabled;
@override
Color? resolve(Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return disabled;
}
return color;
}
}
@immutable
class _OutlinedButtonDefaultOverlay extends MaterialStateProperty<Color?> with Diagnosticable {
_OutlinedButtonDefaultOverlay(this.foreground);
final Color foreground;
@override
Color? resolve(Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return foreground.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return foreground.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return foreground.withOpacity(0.1);
}
return null;
}
}
@immutable
class _OutlinedButtonDefaultMouseCursor extends MaterialStateProperty<MouseCursor?> with Diagnosticable {
_OutlinedButtonDefaultMouseCursor(this.enabledCursor, this.disabledCursor);
final MouseCursor? enabledCursor;
final MouseCursor? disabledCursor;
@override
MouseCursor? resolve(Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return disabledCursor;
}
return enabledCursor;
}
}
class _OutlinedButtonWithIcon extends OutlinedButton {
_OutlinedButtonWithIcon({
super.key,
required super.onPressed,
super.onLongPress,
super.style,
super.focusNode,
bool? autofocus,
super.clipBehavior,
super.statesController,
required Widget icon,
required Widget label,
super.iconAlignment,
}) : super(
autofocus: autofocus ?? false,
child: _OutlinedButtonWithIconChild(
icon: icon,
label: label,
buttonStyle: style,
iconAlignment: iconAlignment,
),
);
@override
ButtonStyle defaultStyleOf(BuildContext context) {
final bool useMaterial3 = Theme.of(context).useMaterial3;
if (!useMaterial3) {
return super.defaultStyleOf(context);
}
final ButtonStyle buttonStyle = super.defaultStyleOf(context);
final double defaultFontSize = buttonStyle.textStyle?.resolve(const <MaterialState>{})?.fontSize ?? 14.0;
final double effectiveTextScale = MediaQuery.textScalerOf(context).scale(defaultFontSize) / 14.0;
final EdgeInsetsGeometry scaledPadding = ButtonStyleButton.scaledPadding(
const EdgeInsetsDirectional.fromSTEB(16, 0, 24, 0),
const EdgeInsetsDirectional.fromSTEB(8, 0, 12, 0),
const EdgeInsetsDirectional.fromSTEB(4, 0, 6, 0),
effectiveTextScale,
);
return buttonStyle.copyWith(
padding: MaterialStatePropertyAll<EdgeInsetsGeometry>(scaledPadding),
);
}
}
class _OutlinedButtonWithIconChild extends StatelessWidget {
const _OutlinedButtonWithIconChild({
required this.label,
required this.icon,
required this.buttonStyle,
required this.iconAlignment,
});
final Widget label;
final Widget icon;
final ButtonStyle? buttonStyle;
final IconAlignment iconAlignment;
@override
Widget build(BuildContext context) {
final double defaultFontSize = buttonStyle?.textStyle?.resolve(const <MaterialState>{})?.fontSize ?? 14.0;
final double scale = clampDouble(MediaQuery.textScalerOf(context).scale(defaultFontSize) / 14.0, 1.0, 2.0) - 1.0;
final double gap = lerpDouble(8, 4, scale)!;
return Row(
mainAxisSize: MainAxisSize.min,
children: iconAlignment == IconAlignment.start
? <Widget>[icon, SizedBox(width: gap), Flexible(child: label)]
: <Widget>[Flexible(child: label), SizedBox(width: gap), icon],
);
}
}
// BEGIN GENERATED TOKEN PROPERTIES - OutlinedButton
// Do not edit by hand. The code between the "BEGIN GENERATED" and
// "END GENERATED" comments are generated from data in the Material
// Design token database by the script:
// dev/tools/gen_defaults/bin/gen_defaults.dart.
class _OutlinedButtonDefaultsM3 extends ButtonStyle {
_OutlinedButtonDefaultsM3(this.context)
: super(
animationDuration: kThemeChangeDuration,
enableFeedback: true,
alignment: Alignment.center,
);
final BuildContext context;
late final ColorScheme _colors = Theme.of(context).colorScheme;
@override
MaterialStateProperty<TextStyle?> get textStyle =>
MaterialStatePropertyAll<TextStyle?>(Theme.of(context).textTheme.labelLarge);
@override
MaterialStateProperty<Color?>? get backgroundColor =>
const MaterialStatePropertyAll<Color>(Colors.transparent);
@override
MaterialStateProperty<Color?>? get foregroundColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return _colors.onSurface.withOpacity(0.38);
}
return _colors.primary;
});
@override
MaterialStateProperty<Color?>? get overlayColor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return _colors.primary.withOpacity(0.1);
}
if (states.contains(MaterialState.hovered)) {
return _colors.primary.withOpacity(0.08);
}
if (states.contains(MaterialState.focused)) {
return _colors.primary.withOpacity(0.1);
}
return null;
});
@override
MaterialStateProperty<Color>? get shadowColor =>
const MaterialStatePropertyAll<Color>(Colors.transparent);
@override
MaterialStateProperty<Color>? get surfaceTintColor =>
const MaterialStatePropertyAll<Color>(Colors.transparent);
@override
MaterialStateProperty<double>? get elevation =>
const MaterialStatePropertyAll<double>(0.0);
@override
MaterialStateProperty<EdgeInsetsGeometry>? get padding =>
MaterialStatePropertyAll<EdgeInsetsGeometry>(_scaledPadding(context));
@override
MaterialStateProperty<Size>? get minimumSize =>
const MaterialStatePropertyAll<Size>(Size(64.0, 40.0));
// No default fixedSize
@override
MaterialStateProperty<Size>? get maximumSize =>
const MaterialStatePropertyAll<Size>(Size.infinite);
@override
MaterialStateProperty<BorderSide>? get side =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return BorderSide(color: _colors.onSurface.withOpacity(0.12));
}
if (states.contains(MaterialState.focused)) {
return BorderSide(color: _colors.primary);
}
return BorderSide(color: _colors.outline);
});
@override
MaterialStateProperty<OutlinedBorder>? get shape =>
const MaterialStatePropertyAll<OutlinedBorder>(StadiumBorder());
@override
MaterialStateProperty<MouseCursor?>? get mouseCursor =>
MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return SystemMouseCursors.basic;
}
return SystemMouseCursors.click;
});
@override
VisualDensity? get visualDensity => Theme.of(context).visualDensity;
@override
MaterialTapTargetSize? get tapTargetSize => Theme.of(context).materialTapTargetSize;
@override
InteractiveInkFeatureFactory? get splashFactory => Theme.of(context).splashFactory;
}
// END GENERATED TOKEN PROPERTIES - OutlinedButton
| flutter/packages/flutter/lib/src/material/outlined_button.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/outlined_button.dart",
"repo_id": "flutter",
"token_count": 7781
} | 655 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/gestures.dart';
import 'color_scheme.dart';
import 'material_state.dart';
import 'scrollbar_theme.dart';
import 'theme.dart';
const double _kScrollbarThickness = 8.0;
const double _kScrollbarThicknessWithTrack = 12.0;
const double _kScrollbarMargin = 2.0;
const double _kScrollbarMinLength = 48.0;
const Radius _kScrollbarRadius = Radius.circular(8.0);
const Duration _kScrollbarFadeDuration = Duration(milliseconds: 300);
const Duration _kScrollbarTimeToFade = Duration(milliseconds: 600);
/// A Material Design scrollbar.
///
/// To add a scrollbar to a [ScrollView], wrap the scroll view
/// widget in a [Scrollbar] widget.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=DbkIQSvwnZc}
///
/// {@macro flutter.widgets.Scrollbar}
///
/// Dynamically changes to a [CupertinoScrollbar], an iOS style scrollbar, by
/// default on the iOS platform.
///
/// The color of the Scrollbar thumb will change when [MaterialState.dragged],
/// or [MaterialState.hovered] on desktop and web platforms. These stateful
/// color choices can be changed using [ScrollbarThemeData.thumbColor].
///
/// {@tool dartpad}
/// This sample shows a [Scrollbar] that executes a fade animation as scrolling
/// occurs. The Scrollbar will fade into view as the user scrolls, and fade out
/// when scrolling stops.
///
/// ** See code in examples/api/lib/material/scrollbar/scrollbar.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// When [thumbVisibility] is true, the scrollbar thumb will remain visible
/// without the fade animation. This requires that a [ScrollController] is
/// provided to controller, or that the [PrimaryScrollController] is available.
///
/// When a [ScrollView.scrollDirection] is [Axis.horizontal], it is recommended
/// that the [Scrollbar] is always visible, since scrolling in the horizontal
/// axis is less discoverable.
///
/// ** See code in examples/api/lib/material/scrollbar/scrollbar.1.dart **
/// {@end-tool}
///
/// A scrollbar track can be added using [trackVisibility]. This can also be
/// drawn when triggered by a hover event, or based on any [MaterialState] by
/// using [ScrollbarThemeData.trackVisibility].
///
/// The [thickness] of the track and scrollbar thumb can be changed dynamically
/// in response to [MaterialState]s using [ScrollbarThemeData.thickness].
///
/// See also:
///
/// * [RawScrollbar], a basic scrollbar that fades in and out, extended
/// by this class to add more animations and behaviors.
/// * [ScrollbarTheme], which configures the Scrollbar's appearance.
/// * [CupertinoScrollbar], an iOS style scrollbar.
/// * [ListView], which displays a linear, scrollable list of children.
/// * [GridView], which displays a 2 dimensional, scrollable array of children.
class Scrollbar extends StatelessWidget {
/// Creates a Material Design scrollbar that by default will connect to the
/// closest Scrollable descendant of [child].
///
/// The [child] should be a source of [ScrollNotification] notifications,
/// typically a [Scrollable] widget.
///
/// If the [controller] is null, the default behavior is to
/// enable scrollbar dragging using the [PrimaryScrollController].
///
/// When null, [thickness] defaults to 8.0 pixels on desktop and web, and 4.0
/// pixels when on mobile platforms. A null [radius] will result in a default
/// of an 8.0 pixel circular radius about the corners of the scrollbar thumb,
/// except for when executing on [TargetPlatform.android], which will render the
/// thumb without a radius.
const Scrollbar({
super.key,
required this.child,
this.controller,
this.thumbVisibility,
this.trackVisibility,
this.thickness,
this.radius,
this.notificationPredicate,
this.interactive,
this.scrollbarOrientation,
});
/// {@macro flutter.widgets.Scrollbar.child}
final Widget child;
/// {@macro flutter.widgets.Scrollbar.controller}
final ScrollController? controller;
/// {@macro flutter.widgets.Scrollbar.thumbVisibility}
///
/// If this property is null, then [ScrollbarThemeData.thumbVisibility] of
/// [ThemeData.scrollbarTheme] is used. If that is also null, the default value
/// is false.
///
/// If the thumb visibility is related to the scrollbar's material state,
/// use the global [ScrollbarThemeData.thumbVisibility] or override the
/// sub-tree's theme data.
final bool? thumbVisibility;
/// {@macro flutter.widgets.Scrollbar.trackVisibility}
///
/// If this property is null, then [ScrollbarThemeData.trackVisibility] of
/// [ThemeData.scrollbarTheme] is used. If that is also null, the default value
/// is false.
///
/// If the track visibility is related to the scrollbar's material state,
/// use the global [ScrollbarThemeData.trackVisibility] or override the
/// sub-tree's theme data.
final bool? trackVisibility;
/// The thickness of the scrollbar in the cross axis of the scrollable.
///
/// If null, the default value is platform dependent. On [TargetPlatform.android],
/// the default thickness is 4.0 pixels. On [TargetPlatform.iOS],
/// [CupertinoScrollbar.defaultThickness] is used. The remaining platforms have a
/// default thickness of 8.0 pixels.
final double? thickness;
/// The [Radius] of the scrollbar thumb's rounded rectangle corners.
///
/// If null, the default value is platform dependent. On [TargetPlatform.android],
/// no radius is applied to the scrollbar thumb. On [TargetPlatform.iOS],
/// [CupertinoScrollbar.defaultRadius] is used. The remaining platforms have a
/// default [Radius.circular] of 8.0 pixels.
final Radius? radius;
/// {@macro flutter.widgets.Scrollbar.interactive}
final bool? interactive;
/// {@macro flutter.widgets.Scrollbar.notificationPredicate}
final ScrollNotificationPredicate? notificationPredicate;
/// {@macro flutter.widgets.Scrollbar.scrollbarOrientation}
final ScrollbarOrientation? scrollbarOrientation;
@override
Widget build(BuildContext context) {
if (Theme.of(context).platform == TargetPlatform.iOS) {
return CupertinoScrollbar(
thumbVisibility: thumbVisibility ?? false,
thickness: thickness ?? CupertinoScrollbar.defaultThickness,
thicknessWhileDragging: thickness ?? CupertinoScrollbar.defaultThicknessWhileDragging,
radius: radius ?? CupertinoScrollbar.defaultRadius,
radiusWhileDragging: radius ?? CupertinoScrollbar.defaultRadiusWhileDragging,
controller: controller,
notificationPredicate: notificationPredicate,
scrollbarOrientation: scrollbarOrientation,
child: child,
);
}
return _MaterialScrollbar(
controller: controller,
thumbVisibility: thumbVisibility,
trackVisibility: trackVisibility,
thickness: thickness,
radius: radius,
notificationPredicate: notificationPredicate,
interactive: interactive,
scrollbarOrientation: scrollbarOrientation,
child: child,
);
}
}
class _MaterialScrollbar extends RawScrollbar {
const _MaterialScrollbar({
required super.child,
super.controller,
super.thumbVisibility,
super.trackVisibility,
super.thickness,
super.radius,
ScrollNotificationPredicate? notificationPredicate,
super.interactive,
super.scrollbarOrientation,
}) : super(
fadeDuration: _kScrollbarFadeDuration,
timeToFade: _kScrollbarTimeToFade,
pressDuration: Duration.zero,
notificationPredicate: notificationPredicate ?? defaultScrollNotificationPredicate,
);
@override
_MaterialScrollbarState createState() => _MaterialScrollbarState();
}
class _MaterialScrollbarState extends RawScrollbarState<_MaterialScrollbar> {
late AnimationController _hoverAnimationController;
bool _dragIsActive = false;
bool _hoverIsActive = false;
late ColorScheme _colorScheme;
late ScrollbarThemeData _scrollbarTheme;
// On Android, scrollbars should match native appearance.
late bool _useAndroidScrollbar;
@override
bool get showScrollbar => widget.thumbVisibility ?? _scrollbarTheme.thumbVisibility?.resolve(_states) ?? false;
@override
bool get enableGestures => widget.interactive ?? _scrollbarTheme.interactive ?? !_useAndroidScrollbar;
MaterialStateProperty<bool> get _trackVisibility => MaterialStateProperty.resolveWith((Set<MaterialState> states) {
return widget.trackVisibility ?? _scrollbarTheme.trackVisibility?.resolve(states) ?? false;
});
Set<MaterialState> get _states => <MaterialState>{
if (_dragIsActive) MaterialState.dragged,
if (_hoverIsActive) MaterialState.hovered,
};
MaterialStateProperty<Color> get _thumbColor {
final Color onSurface = _colorScheme.onSurface;
final Brightness brightness = _colorScheme.brightness;
late Color dragColor;
late Color hoverColor;
late Color idleColor;
switch (brightness) {
case Brightness.light:
dragColor = onSurface.withOpacity(0.6);
hoverColor = onSurface.withOpacity(0.5);
idleColor = _useAndroidScrollbar
? Theme.of(context).highlightColor.withOpacity(1.0)
: onSurface.withOpacity(0.1);
case Brightness.dark:
dragColor = onSurface.withOpacity(0.75);
hoverColor = onSurface.withOpacity(0.65);
idleColor = _useAndroidScrollbar
? Theme.of(context).highlightColor.withOpacity(1.0)
: onSurface.withOpacity(0.3);
}
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.dragged)) {
return _scrollbarTheme.thumbColor?.resolve(states) ?? dragColor;
}
// If the track is visible, the thumb color hover animation is ignored and
// changes immediately.
if (_trackVisibility.resolve(states)) {
return _scrollbarTheme.thumbColor?.resolve(states) ?? hoverColor;
}
return Color.lerp(
_scrollbarTheme.thumbColor?.resolve(states) ?? idleColor,
_scrollbarTheme.thumbColor?.resolve(states) ?? hoverColor,
_hoverAnimationController.value,
)!;
});
}
MaterialStateProperty<Color> get _trackColor {
final Color onSurface = _colorScheme.onSurface;
final Brightness brightness = _colorScheme.brightness;
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (showScrollbar && _trackVisibility.resolve(states)) {
return _scrollbarTheme.trackColor?.resolve(states)
?? (brightness == Brightness.light
? onSurface.withOpacity(0.03)
: onSurface.withOpacity(0.05));
}
return const Color(0x00000000);
});
}
MaterialStateProperty<Color> get _trackBorderColor {
final Color onSurface = _colorScheme.onSurface;
final Brightness brightness = _colorScheme.brightness;
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (showScrollbar && _trackVisibility.resolve(states)) {
return _scrollbarTheme.trackBorderColor?.resolve(states)
?? (brightness == Brightness.light
? onSurface.withOpacity(0.1)
: onSurface.withOpacity(0.25));
}
return const Color(0x00000000);
});
}
MaterialStateProperty<double> get _thickness {
return MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.hovered) && _trackVisibility.resolve(states)) {
return widget.thickness
?? _scrollbarTheme.thickness?.resolve(states)
?? _kScrollbarThicknessWithTrack;
}
// The default scrollbar thickness is smaller on mobile.
return widget.thickness
?? _scrollbarTheme.thickness?.resolve(states)
?? (_kScrollbarThickness / (_useAndroidScrollbar ? 2 : 1));
});
}
@override
void initState() {
super.initState();
_hoverAnimationController = AnimationController(
vsync: this,
duration: const Duration(milliseconds: 200),
);
_hoverAnimationController.addListener(() {
updateScrollbarPainter();
});
}
@override
void didChangeDependencies() {
final ThemeData theme = Theme.of(context);
_colorScheme = theme.colorScheme;
_scrollbarTheme = ScrollbarTheme.of(context);
switch (theme.platform) {
case TargetPlatform.android:
_useAndroidScrollbar = true;
case TargetPlatform.iOS:
case TargetPlatform.linux:
case TargetPlatform.fuchsia:
case TargetPlatform.macOS:
case TargetPlatform.windows:
_useAndroidScrollbar = false;
}
super.didChangeDependencies();
}
@override
void updateScrollbarPainter() {
scrollbarPainter
..color = _thumbColor.resolve(_states)
..trackColor = _trackColor.resolve(_states)
..trackBorderColor = _trackBorderColor.resolve(_states)
..textDirection = Directionality.of(context)
..thickness = _thickness.resolve(_states)
..radius = widget.radius ?? _scrollbarTheme.radius ?? (_useAndroidScrollbar ? null : _kScrollbarRadius)
..crossAxisMargin = _scrollbarTheme.crossAxisMargin ?? (_useAndroidScrollbar ? 0.0 : _kScrollbarMargin)
..mainAxisMargin = _scrollbarTheme.mainAxisMargin ?? 0.0
..minLength = _scrollbarTheme.minThumbLength ?? _kScrollbarMinLength
..padding = MediaQuery.paddingOf(context)
..scrollbarOrientation = widget.scrollbarOrientation
..ignorePointer = !enableGestures;
}
@override
void handleThumbPressStart(Offset localPosition) {
super.handleThumbPressStart(localPosition);
setState(() { _dragIsActive = true; });
}
@override
void handleThumbPressEnd(Offset localPosition, Velocity velocity) {
super.handleThumbPressEnd(localPosition, velocity);
setState(() { _dragIsActive = false; });
}
@override
void handleHover(PointerHoverEvent event) {
super.handleHover(event);
// Check if the position of the pointer falls over the painted scrollbar
if (isPointerOverScrollbar(event.position, event.kind, forHover: true)) {
// Pointer is hovering over the scrollbar
setState(() { _hoverIsActive = true; });
_hoverAnimationController.forward();
} else if (_hoverIsActive) {
// Pointer was, but is no longer over painted scrollbar.
setState(() { _hoverIsActive = false; });
_hoverAnimationController.reverse();
}
}
@override
void handleHoverExit(PointerExitEvent event) {
super.handleHoverExit(event);
setState(() { _hoverIsActive = false; });
_hoverAnimationController.reverse();
}
@override
void dispose() {
_hoverAnimationController.dispose();
super.dispose();
}
}
| flutter/packages/flutter/lib/src/material/scrollbar.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/scrollbar.dart",
"repo_id": "flutter",
"token_count": 4939
} | 656 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter/services.dart' show SelectionChangedCause, SuggestionSpan;
import 'adaptive_text_selection_toolbar.dart';
import 'colors.dart';
import 'material.dart';
import 'spell_check_suggestions_toolbar_layout_delegate.dart';
import 'text_selection_toolbar_text_button.dart';
// The default height of the SpellCheckSuggestionsToolbar, which
// assumes there are the maximum number of spell check suggestions available, 3.
// Size eyeballed on Pixel 4 emulator running Android API 31.
const double _kDefaultToolbarHeight = 193.0;
/// The maximum number of suggestions in the toolbar is 3, plus a delete button.
const int _kMaxSuggestions = 3;
/// The default spell check suggestions toolbar for Android.
///
/// Tries to position itself below the [anchor], but if it doesn't fit, then it
/// readjusts to fit above bottom view insets.
///
/// See also:
///
/// * [CupertinoSpellCheckSuggestionsToolbar], which is similar but builds an
/// iOS-style spell check toolbar.
class SpellCheckSuggestionsToolbar extends StatelessWidget {
/// Constructs a [SpellCheckSuggestionsToolbar].
///
/// [buttonItems] must not contain more than four items, generally three
/// suggestions and one delete button.
const SpellCheckSuggestionsToolbar({
super.key,
required this.anchor,
required this.buttonItems,
}) : assert(buttonItems.length <= _kMaxSuggestions + 1);
/// Constructs a [SpellCheckSuggestionsToolbar] with the default children for
/// an [EditableText].
///
/// See also:
/// * [CupertinoSpellCheckSuggestionsToolbar.editableText], which is similar
/// but builds an iOS-style toolbar.
SpellCheckSuggestionsToolbar.editableText({
super.key,
required EditableTextState editableTextState,
}) : buttonItems = buildButtonItems(editableTextState) ?? <ContextMenuButtonItem>[],
anchor = getToolbarAnchor(editableTextState.contextMenuAnchors);
/// {@template flutter.material.SpellCheckSuggestionsToolbar.anchor}
/// The focal point below which the toolbar attempts to position itself.
/// {@endtemplate}
final Offset anchor;
/// The [ContextMenuButtonItem]s that will be turned into the correct button
/// widgets and displayed in the spell check suggestions toolbar.
///
/// Must not contain more than four items, typically three suggestions and a
/// delete button.
///
/// See also:
///
/// * [AdaptiveTextSelectionToolbar.buttonItems], the list of
/// [ContextMenuButtonItem]s that are used to build the buttons of the
/// text selection toolbar.
/// * [CupertinoSpellCheckSuggestionsToolbar.buttonItems], the list of
/// [ContextMenuButtonItem]s used to build the Cupertino style spell check
/// suggestions toolbar.
final List<ContextMenuButtonItem> buttonItems;
/// Builds the button items for the toolbar based on the available
/// spell check suggestions.
static List<ContextMenuButtonItem>? buildButtonItems(
EditableTextState editableTextState,
) {
// Determine if composing region is misspelled.
final SuggestionSpan? spanAtCursorIndex =
editableTextState.findSuggestionSpanAtCursorIndex(
editableTextState.currentTextEditingValue.selection.baseOffset,
);
if (spanAtCursorIndex == null) {
return null;
}
final List<ContextMenuButtonItem> buttonItems = <ContextMenuButtonItem>[];
// Build suggestion buttons.
for (final String suggestion in spanAtCursorIndex.suggestions.take(_kMaxSuggestions)) {
buttonItems.add(ContextMenuButtonItem(
onPressed: () {
if (!editableTextState.mounted) {
return;
}
_replaceText(
editableTextState,
suggestion,
spanAtCursorIndex.range,
);
},
label: suggestion,
));
}
// Build delete button.
final ContextMenuButtonItem deleteButton =
ContextMenuButtonItem(
onPressed: () {
if (!editableTextState.mounted) {
return;
}
_replaceText(
editableTextState,
'',
editableTextState.currentTextEditingValue.composing,
);
},
type: ContextMenuButtonType.delete,
);
buttonItems.add(deleteButton);
return buttonItems;
}
static void _replaceText(EditableTextState editableTextState, String text, TextRange replacementRange) {
// Replacement cannot be performed if the text is read only or obscured.
assert(!editableTextState.widget.readOnly && !editableTextState.widget.obscureText);
final TextEditingValue newValue = editableTextState.textEditingValue.replaced(
replacementRange,
text,
);
editableTextState.userUpdateTextEditingValue(newValue, SelectionChangedCause.toolbar);
// Schedule a call to bringIntoView() after renderEditable updates.
SchedulerBinding.instance.addPostFrameCallback((Duration duration) {
if (editableTextState.mounted) {
editableTextState.bringIntoView(editableTextState.textEditingValue.selection.extent);
}
}, debugLabel: 'SpellCheckerSuggestionsToolbar.bringIntoView');
editableTextState.hideToolbar();
}
/// Determines the Offset that the toolbar will be anchored to.
static Offset getToolbarAnchor(TextSelectionToolbarAnchors anchors) {
// Since this will be positioned below the anchor point, use the secondary
// anchor by default.
return anchors.secondaryAnchor == null ? anchors.primaryAnchor : anchors.secondaryAnchor!;
}
/// Builds the toolbar buttons based on the [buttonItems].
List<Widget> _buildToolbarButtons(BuildContext context) {
return buttonItems.map((ContextMenuButtonItem buttonItem) {
final TextSelectionToolbarTextButton button =
TextSelectionToolbarTextButton(
padding: const EdgeInsets.fromLTRB(20, 0, 0, 0),
onPressed: buttonItem.onPressed,
alignment: Alignment.centerLeft,
child: Text(
AdaptiveTextSelectionToolbar.getButtonLabel(context, buttonItem),
style: buttonItem.type == ContextMenuButtonType.delete ? const TextStyle(color: Colors.blue) : null,
),
);
if (buttonItem.type != ContextMenuButtonType.delete) {
return button;
}
return DecoratedBox(
decoration: const BoxDecoration(border: Border(top: BorderSide(color: Colors.grey))),
child: button,
);
}).toList();
}
@override
Widget build(BuildContext context) {
if (buttonItems.isEmpty){
return const SizedBox.shrink();
}
// Adjust toolbar height if needed.
final double spellCheckSuggestionsToolbarHeight =
_kDefaultToolbarHeight - (48.0 * (4 - buttonItems.length));
// Incorporate the padding distance between the content and toolbar.
final MediaQueryData mediaQueryData = MediaQuery.of(context);
final double softKeyboardViewInsetsBottom = mediaQueryData.viewInsets.bottom;
final double paddingAbove = mediaQueryData.padding.top
+ CupertinoTextSelectionToolbar.kToolbarScreenPadding;
// Makes up for the Padding.
final Offset localAdjustment = Offset(
CupertinoTextSelectionToolbar.kToolbarScreenPadding,
paddingAbove,
);
return Padding(
padding: EdgeInsets.fromLTRB(
CupertinoTextSelectionToolbar.kToolbarScreenPadding,
paddingAbove,
CupertinoTextSelectionToolbar.kToolbarScreenPadding,
CupertinoTextSelectionToolbar.kToolbarScreenPadding + softKeyboardViewInsetsBottom,
),
child: CustomSingleChildLayout(
delegate: SpellCheckSuggestionsToolbarLayoutDelegate(
anchor: anchor - localAdjustment,
),
child: AnimatedSize(
// This duration was eyeballed on a Pixel 2 emulator running Android
// API 28 for the Material TextSelectionToolbar.
duration: const Duration(milliseconds: 140),
child: _SpellCheckSuggestionsToolbarContainer(
height: spellCheckSuggestionsToolbarHeight,
children: <Widget>[..._buildToolbarButtons(context)],
),
),
),
);
}
}
/// The Material-styled toolbar outline for the spell check suggestions
/// toolbar.
class _SpellCheckSuggestionsToolbarContainer extends StatelessWidget {
const _SpellCheckSuggestionsToolbarContainer({
required this.height,
required this.children,
});
final double height;
final List<Widget> children;
@override
Widget build(BuildContext context) {
return Material(
// This elevation was eyeballed on a Pixel 4 emulator running Android
// API 31 for the SpellCheckSuggestionsToolbar.
elevation: 2.0,
type: MaterialType.card,
child: SizedBox(
// This width was eyeballed on a Pixel 4 emulator running Android
// API 31 for the SpellCheckSuggestionsToolbar.
width: 165.0,
height: height,
child: Column(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: children,
),
),
);
}
}
| flutter/packages/flutter/lib/src/material/spell_check_suggestions_toolbar.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/spell_check_suggestions_toolbar.dart",
"repo_id": "flutter",
"token_count": 3217
} | 657 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart' show listEquals;
import 'package:flutter/rendering.dart';
import 'color_scheme.dart';
import 'debug.dart';
import 'icon_button.dart';
import 'icons.dart';
import 'material.dart';
import 'material_localizations.dart';
import 'theme.dart';
const double _kToolbarHeight = 44.0;
const double _kToolbarContentDistance = 8.0;
/// A fully-functional Material-style text selection toolbar.
///
/// Tries to position itself above [anchorAbove], but if it doesn't fit, then
/// it positions itself below [anchorBelow].
///
/// If any children don't fit in the menu, an overflow menu will automatically
/// be created.
///
/// See also:
///
/// * [AdaptiveTextSelectionToolbar], which builds the toolbar for the current
/// platform.
/// * [CupertinoTextSelectionToolbar], which is similar, but builds an iOS-
/// style toolbar.
class TextSelectionToolbar extends StatelessWidget {
/// Creates an instance of TextSelectionToolbar.
const TextSelectionToolbar({
super.key,
required this.anchorAbove,
required this.anchorBelow,
this.toolbarBuilder = _defaultToolbarBuilder,
required this.children,
}) : assert(children.length > 0);
/// {@template flutter.material.TextSelectionToolbar.anchorAbove}
/// The focal point above which the toolbar attempts to position itself.
///
/// If there is not enough room above before reaching the top of the screen,
/// then the toolbar will position itself below [anchorBelow].
/// {@endtemplate}
final Offset anchorAbove;
/// {@template flutter.material.TextSelectionToolbar.anchorBelow}
/// The focal point below which the toolbar attempts to position itself, if it
/// doesn't fit above [anchorAbove].
/// {@endtemplate}
final Offset anchorBelow;
/// {@template flutter.material.TextSelectionToolbar.children}
/// The children that will be displayed in the text selection toolbar.
///
/// Typically these are buttons.
///
/// Must not be empty.
/// {@endtemplate}
///
/// See also:
/// * [TextSelectionToolbarTextButton], which builds a default Material-
/// style text selection toolbar text button.
final List<Widget> children;
/// {@template flutter.material.TextSelectionToolbar.toolbarBuilder}
/// Builds the toolbar container.
///
/// Useful for customizing the high-level background of the toolbar. The given
/// child Widget will contain all of the [children].
/// {@endtemplate}
final ToolbarBuilder toolbarBuilder;
/// The size of the text selection handles.
///
/// See also:
///
/// * [SpellCheckSuggestionsToolbar], which references this value to calculate
/// the padding between the toolbar and anchor.
static const double kHandleSize = 22.0;
/// Padding between the toolbar and the anchor.
static const double kToolbarContentDistanceBelow = kHandleSize - 2.0;
// Build the default Android Material text selection menu toolbar.
static Widget _defaultToolbarBuilder(BuildContext context, Widget child) {
return _TextSelectionToolbarContainer(
child: child,
);
}
@override
Widget build(BuildContext context) {
// Incorporate the padding distance between the content and toolbar.
final Offset anchorAbovePadded =
anchorAbove - const Offset(0.0, _kToolbarContentDistance);
final Offset anchorBelowPadded =
anchorBelow + const Offset(0.0, kToolbarContentDistanceBelow);
const double screenPadding = CupertinoTextSelectionToolbar.kToolbarScreenPadding;
final double paddingAbove = MediaQuery.paddingOf(context).top
+ screenPadding;
final double availableHeight = anchorAbovePadded.dy - _kToolbarContentDistance - paddingAbove;
final bool fitsAbove = _kToolbarHeight <= availableHeight;
// Makes up for the Padding above the Stack.
final Offset localAdjustment = Offset(screenPadding, paddingAbove);
return Padding(
padding: EdgeInsets.fromLTRB(
screenPadding,
paddingAbove,
screenPadding,
screenPadding,
),
child: CustomSingleChildLayout(
delegate: TextSelectionToolbarLayoutDelegate(
anchorAbove: anchorAbovePadded - localAdjustment,
anchorBelow: anchorBelowPadded - localAdjustment,
fitsAbove: fitsAbove,
),
child: _TextSelectionToolbarOverflowable(
isAbove: fitsAbove,
toolbarBuilder: toolbarBuilder,
children: children,
),
),
);
}
}
// A toolbar containing the given children. If they overflow the width
// available, then the overflowing children will be displayed in an overflow
// menu.
class _TextSelectionToolbarOverflowable extends StatefulWidget {
const _TextSelectionToolbarOverflowable({
required this.isAbove,
required this.toolbarBuilder,
required this.children,
}) : assert(children.length > 0);
final List<Widget> children;
// When true, the toolbar fits above its anchor and will be positioned there.
final bool isAbove;
// Builds the toolbar that will be populated with the children and fit inside
// of the layout that adjusts to overflow.
final ToolbarBuilder toolbarBuilder;
@override
_TextSelectionToolbarOverflowableState createState() => _TextSelectionToolbarOverflowableState();
}
class _TextSelectionToolbarOverflowableState extends State<_TextSelectionToolbarOverflowable> with TickerProviderStateMixin {
// Whether or not the overflow menu is open. When it is closed, the menu
// items that don't overflow are shown. When it is open, only the overflowing
// menu items are shown.
bool _overflowOpen = false;
// The key for _TextSelectionToolbarTrailingEdgeAlign.
UniqueKey _containerKey = UniqueKey();
// Close the menu and reset layout calculations, as in when the menu has
// changed and saved values are no longer relevant. This should be called in
// setState or another context where a rebuild is happening.
void _reset() {
// Change _TextSelectionToolbarTrailingEdgeAlign's key when the menu changes
// in order to cause it to rebuild. This lets it recalculate its
// saved width for the new set of children, and it prevents AnimatedSize
// from animating the size change.
_containerKey = UniqueKey();
// If the menu items change, make sure the overflow menu is closed. This
// prevents getting into a broken state where _overflowOpen is true when
// there are not enough children to cause overflow.
_overflowOpen = false;
}
@override
void didUpdateWidget(_TextSelectionToolbarOverflowable oldWidget) {
super.didUpdateWidget(oldWidget);
// If the children are changing at all, the current page should be reset.
if (!listEquals(widget.children, oldWidget.children)) {
_reset();
}
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterialLocalizations(context));
final MaterialLocalizations localizations = MaterialLocalizations.of(context);
return _TextSelectionToolbarTrailingEdgeAlign(
key: _containerKey,
overflowOpen: _overflowOpen,
textDirection: Directionality.of(context),
child: AnimatedSize(
// This duration was eyeballed on a Pixel 2 emulator running Android
// API 28.
duration: const Duration(milliseconds: 140),
child: widget.toolbarBuilder(context, _TextSelectionToolbarItemsLayout(
isAbove: widget.isAbove,
overflowOpen: _overflowOpen,
children: <Widget>[
// TODO(justinmc): This overflow button should have its own slot in
// _TextSelectionToolbarItemsLayout separate from children, similar
// to how it's done in Cupertino's text selection menu.
// https://github.com/flutter/flutter/issues/69908
// The navButton that shows and hides the overflow menu is the
// first child.
_TextSelectionToolbarOverflowButton(
icon: Icon(_overflowOpen ? Icons.arrow_back : Icons.more_vert),
onPressed: () {
setState(() {
_overflowOpen = !_overflowOpen;
});
},
tooltip: _overflowOpen
? localizations.backButtonTooltip
: localizations.moreButtonTooltip,
),
...widget.children,
],
)),
),
);
}
}
// When the overflow menu is open, it tries to align its trailing edge to the
// trailing edge of the closed menu. This widget handles this effect by
// measuring and maintaining the width of the closed menu and aligning the child
// to that side.
class _TextSelectionToolbarTrailingEdgeAlign extends SingleChildRenderObjectWidget {
const _TextSelectionToolbarTrailingEdgeAlign({
super.key,
required Widget super.child,
required this.overflowOpen,
required this.textDirection,
});
final bool overflowOpen;
final TextDirection textDirection;
@override
_TextSelectionToolbarTrailingEdgeAlignRenderBox createRenderObject(BuildContext context) {
return _TextSelectionToolbarTrailingEdgeAlignRenderBox(
overflowOpen: overflowOpen,
textDirection: textDirection,
);
}
@override
void updateRenderObject(BuildContext context, _TextSelectionToolbarTrailingEdgeAlignRenderBox renderObject) {
renderObject
..overflowOpen = overflowOpen
..textDirection = textDirection;
}
}
class _TextSelectionToolbarTrailingEdgeAlignRenderBox extends RenderProxyBox {
_TextSelectionToolbarTrailingEdgeAlignRenderBox({
required bool overflowOpen,
required TextDirection textDirection,
}) : _textDirection = textDirection,
_overflowOpen = overflowOpen,
super();
// The width of the menu when it was closed. This is used to achieve the
// behavior where the open menu aligns its trailing edge to the closed menu's
// trailing edge.
double? _closedWidth;
bool _overflowOpen;
bool get overflowOpen => _overflowOpen;
set overflowOpen(bool value) {
if (value == overflowOpen) {
return;
}
_overflowOpen = value;
markNeedsLayout();
}
TextDirection _textDirection;
TextDirection get textDirection => _textDirection;
set textDirection(TextDirection value) {
if (value == textDirection) {
return;
}
_textDirection = value;
markNeedsLayout();
}
@override
void performLayout() {
child!.layout(constraints.loosen(), parentUsesSize: true);
// Save the width when the menu is closed. If the menu changes, this width
// is invalid, so it's important that this RenderBox be recreated in that
// case. Currently, this is achieved by providing a new key to
// _TextSelectionToolbarTrailingEdgeAlign.
if (!overflowOpen && _closedWidth == null) {
_closedWidth = child!.size.width;
}
size = constraints.constrain(Size(
// If the open menu is wider than the closed menu, just use its own width
// and don't worry about aligning the trailing edges.
// _closedWidth is used even when the menu is closed to allow it to
// animate its size while keeping the same edge alignment.
_closedWidth == null || child!.size.width > _closedWidth! ? child!.size.width : _closedWidth!,
child!.size.height,
));
// Set the offset in the parent data such that the child will be aligned to
// the trailing edge, depending on the text direction.
final ToolbarItemsParentData childParentData = child!.parentData! as ToolbarItemsParentData;
childParentData.offset = Offset(
textDirection == TextDirection.rtl ? 0.0 : size.width - child!.size.width,
0.0,
);
}
// Paint at the offset set in the parent data.
@override
void paint(PaintingContext context, Offset offset) {
final ToolbarItemsParentData childParentData = child!.parentData! as ToolbarItemsParentData;
context.paintChild(child!, childParentData.offset + offset);
}
// Include the parent data offset in the hit test.
@override
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
// The x, y parameters have the top left of the node's box as the origin.
final ToolbarItemsParentData childParentData = child!.parentData! as ToolbarItemsParentData;
return result.addWithPaintOffset(
offset: childParentData.offset,
position: position,
hitTest: (BoxHitTestResult result, Offset transformed) {
assert(transformed == position - childParentData.offset);
return child!.hitTest(result, position: transformed);
},
);
}
@override
void setupParentData(RenderBox child) {
if (child.parentData is! ToolbarItemsParentData) {
child.parentData = ToolbarItemsParentData();
}
}
@override
void applyPaintTransform(RenderObject child, Matrix4 transform) {
final ToolbarItemsParentData childParentData = child.parentData! as ToolbarItemsParentData;
transform.translate(childParentData.offset.dx, childParentData.offset.dy);
super.applyPaintTransform(child, transform);
}
}
// Renders the menu items in the correct positions in the menu and its overflow
// submenu based on calculating which item would first overflow.
class _TextSelectionToolbarItemsLayout extends MultiChildRenderObjectWidget {
const _TextSelectionToolbarItemsLayout({
required this.isAbove,
required this.overflowOpen,
required super.children,
});
final bool isAbove;
final bool overflowOpen;
@override
_RenderTextSelectionToolbarItemsLayout createRenderObject(BuildContext context) {
return _RenderTextSelectionToolbarItemsLayout(
isAbove: isAbove,
overflowOpen: overflowOpen,
);
}
@override
void updateRenderObject(BuildContext context, _RenderTextSelectionToolbarItemsLayout renderObject) {
renderObject
..isAbove = isAbove
..overflowOpen = overflowOpen;
}
@override
_TextSelectionToolbarItemsLayoutElement createElement() => _TextSelectionToolbarItemsLayoutElement(this);
}
class _TextSelectionToolbarItemsLayoutElement extends MultiChildRenderObjectElement {
_TextSelectionToolbarItemsLayoutElement(
super.widget,
);
static bool _shouldPaint(Element child) {
return (child.renderObject!.parentData! as ToolbarItemsParentData).shouldPaint;
}
@override
void debugVisitOnstageChildren(ElementVisitor visitor) {
children.where(_shouldPaint).forEach(visitor);
}
}
class _RenderTextSelectionToolbarItemsLayout extends RenderBox with ContainerRenderObjectMixin<RenderBox, ToolbarItemsParentData> {
_RenderTextSelectionToolbarItemsLayout({
required bool isAbove,
required bool overflowOpen,
}) : _isAbove = isAbove,
_overflowOpen = overflowOpen,
super();
// The index of the last item that doesn't overflow.
int _lastIndexThatFits = -1;
bool _isAbove;
bool get isAbove => _isAbove;
set isAbove(bool value) {
if (value == isAbove) {
return;
}
_isAbove = value;
markNeedsLayout();
}
bool _overflowOpen;
bool get overflowOpen => _overflowOpen;
set overflowOpen(bool value) {
if (value == overflowOpen) {
return;
}
_overflowOpen = value;
markNeedsLayout();
}
// Layout the necessary children, and figure out where the children first
// overflow, if at all.
void _layoutChildren() {
// When overflow is not open, the toolbar is always a specific height.
final BoxConstraints sizedConstraints = _overflowOpen
? constraints
: BoxConstraints.loose(Size(
constraints.maxWidth,
_kToolbarHeight,
));
int i = -1;
double width = 0.0;
visitChildren((RenderObject renderObjectChild) {
i++;
// No need to layout children inside the overflow menu when it's closed.
// The opposite is not true. It is necessary to layout the children that
// don't overflow when the overflow menu is open in order to calculate
// _lastIndexThatFits.
if (_lastIndexThatFits != -1 && !overflowOpen) {
return;
}
final RenderBox child = renderObjectChild as RenderBox;
child.layout(sizedConstraints.loosen(), parentUsesSize: true);
width += child.size.width;
if (width > sizedConstraints.maxWidth && _lastIndexThatFits == -1) {
_lastIndexThatFits = i - 1;
}
});
// If the last child overflows, but only because of the width of the
// overflow button, then just show it and hide the overflow button.
final RenderBox navButton = firstChild!;
if (_lastIndexThatFits != -1
&& _lastIndexThatFits == childCount - 2
&& width - navButton.size.width <= sizedConstraints.maxWidth) {
_lastIndexThatFits = -1;
}
}
// Returns true when the child should be painted, false otherwise.
bool _shouldPaintChild(RenderObject renderObjectChild, int index) {
// Paint the navButton when there is overflow.
if (renderObjectChild == firstChild) {
return _lastIndexThatFits != -1;
}
// If there is no overflow, all children besides the navButton are painted.
if (_lastIndexThatFits == -1) {
return true;
}
// When there is overflow, paint if the child is in the part of the menu
// that is currently open. Overflowing children are painted when the
// overflow menu is open, and the children that fit are painted when the
// overflow menu is closed.
return (index > _lastIndexThatFits) == overflowOpen;
}
// Decide which children will be painted, set their shouldPaint, and set the
// offset that painted children will be placed at.
void _placeChildren() {
int i = -1;
Size nextSize = Size.zero;
double fitWidth = 0.0;
final RenderBox navButton = firstChild!;
double overflowHeight = overflowOpen && !isAbove ? navButton.size.height : 0.0;
visitChildren((RenderObject renderObjectChild) {
i++;
final RenderBox child = renderObjectChild as RenderBox;
final ToolbarItemsParentData childParentData = child.parentData! as ToolbarItemsParentData;
// Handle placing the navigation button after iterating all children.
if (renderObjectChild == navButton) {
return;
}
// There is no need to place children that won't be painted.
if (!_shouldPaintChild(renderObjectChild, i)) {
childParentData.shouldPaint = false;
return;
}
childParentData.shouldPaint = true;
if (!overflowOpen) {
childParentData.offset = Offset(fitWidth, 0.0);
fitWidth += child.size.width;
nextSize = Size(
fitWidth,
math.max(child.size.height, nextSize.height),
);
} else {
childParentData.offset = Offset(0.0, overflowHeight);
overflowHeight += child.size.height;
nextSize = Size(
math.max(child.size.width, nextSize.width),
overflowHeight,
);
}
});
// Place the navigation button if needed.
final ToolbarItemsParentData navButtonParentData = navButton.parentData! as ToolbarItemsParentData;
if (_shouldPaintChild(firstChild!, 0)) {
navButtonParentData.shouldPaint = true;
if (overflowOpen) {
navButtonParentData.offset = isAbove
? Offset(0.0, overflowHeight)
: Offset.zero;
nextSize = Size(
nextSize.width,
isAbove ? nextSize.height + navButton.size.height : nextSize.height,
);
} else {
navButtonParentData.offset = Offset(fitWidth, 0.0);
nextSize = Size(nextSize.width + navButton.size.width, nextSize.height);
}
} else {
navButtonParentData.shouldPaint = false;
}
size = nextSize;
}
// Horizontally expand the children when the menu overflows so they can react to
// pointer events into their whole area.
void _resizeChildrenWhenOverflow() {
if (!overflowOpen) {
return;
}
final RenderBox navButton = firstChild!;
int i = -1;
visitChildren((RenderObject renderObjectChild) {
final RenderBox child = renderObjectChild as RenderBox;
final ToolbarItemsParentData childParentData = child.parentData! as ToolbarItemsParentData;
i++;
// Ignore the navigation button.
if (renderObjectChild == navButton) {
return;
}
// There is no need to update children that won't be painted.
if (!_shouldPaintChild(renderObjectChild, i)) {
childParentData.shouldPaint = false;
return;
}
child.layout(
BoxConstraints.tightFor(width: size.width),
parentUsesSize: true,
);
});
}
@override
void performLayout() {
_lastIndexThatFits = -1;
if (firstChild == null) {
size = constraints.smallest;
return;
}
_layoutChildren();
_placeChildren();
_resizeChildrenWhenOverflow();
}
@override
void paint(PaintingContext context, Offset offset) {
visitChildren((RenderObject renderObjectChild) {
final RenderBox child = renderObjectChild as RenderBox;
final ToolbarItemsParentData childParentData = child.parentData! as ToolbarItemsParentData;
if (!childParentData.shouldPaint) {
return;
}
context.paintChild(child, childParentData.offset + offset);
});
}
@override
void setupParentData(RenderBox child) {
if (child.parentData is! ToolbarItemsParentData) {
child.parentData = ToolbarItemsParentData();
}
}
@override
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
RenderBox? child = lastChild;
while (child != null) {
// The x, y parameters have the top left of the node's box as the origin.
final ToolbarItemsParentData childParentData = child.parentData! as ToolbarItemsParentData;
// Don't hit test children aren't shown.
if (!childParentData.shouldPaint) {
child = childParentData.previousSibling;
continue;
}
final bool isHit = result.addWithPaintOffset(
offset: childParentData.offset,
position: position,
hitTest: (BoxHitTestResult result, Offset transformed) {
assert(transformed == position - childParentData.offset);
return child!.hitTest(result, position: transformed);
},
);
if (isHit) {
return true;
}
child = childParentData.previousSibling;
}
return false;
}
// Visit only the children that should be painted.
@override
void visitChildrenForSemantics(RenderObjectVisitor visitor) {
visitChildren((RenderObject renderObjectChild) {
final RenderBox child = renderObjectChild as RenderBox;
final ToolbarItemsParentData childParentData = child.parentData! as ToolbarItemsParentData;
if (childParentData.shouldPaint) {
visitor(renderObjectChild);
}
});
}
}
// The Material-styled toolbar outline. Fill it with any widgets you want. No
// overflow ability.
class _TextSelectionToolbarContainer extends StatelessWidget {
const _TextSelectionToolbarContainer({
required this.child,
});
final Widget child;
// These colors were taken from a screenshot of a Pixel 6 emulator running
// Android API level 34.
static const Color _defaultColorLight = Color(0xffffffff);
static const Color _defaultColorDark = Color(0xff424242);
static Color _getColor(ColorScheme colorScheme) {
final bool isDefaultSurface = switch (colorScheme.brightness) {
Brightness.light => identical(ThemeData().colorScheme.surface, colorScheme.surface),
Brightness.dark => identical(ThemeData.dark().colorScheme.surface, colorScheme.surface),
};
if (!isDefaultSurface) {
return colorScheme.surface;
}
return switch (colorScheme.brightness) {
Brightness.light => _defaultColorLight,
Brightness.dark => _defaultColorDark,
};
}
@override
Widget build(BuildContext context) {
final ThemeData theme = Theme.of(context);
return Material(
// This value was eyeballed to match the native text selection menu on
// a Pixel 6 emulator running Android API level 34.
borderRadius: const BorderRadius.all(Radius.circular(_kToolbarHeight / 2)),
clipBehavior: Clip.antiAlias,
color: _getColor(theme.colorScheme),
elevation: 1.0,
type: MaterialType.card,
child: child,
);
}
}
// A button styled like a Material native Android text selection overflow menu
// forward and back controls.
class _TextSelectionToolbarOverflowButton extends StatelessWidget {
const _TextSelectionToolbarOverflowButton({
required this.icon,
this.onPressed,
this.tooltip,
});
final Icon icon;
final VoidCallback? onPressed;
final String? tooltip;
@override
Widget build(BuildContext context) {
return Material(
type: MaterialType.card,
color: const Color(0x00000000),
child: IconButton(
// TODO(justinmc): This should be an AnimatedIcon, but
// AnimatedIcons doesn't yet support arrow_back to more_vert.
// https://github.com/flutter/flutter/issues/51209
icon: icon,
onPressed: onPressed,
tooltip: tooltip,
),
);
}
}
| flutter/packages/flutter/lib/src/material/text_selection_toolbar.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/material/text_selection_toolbar.dart",
"repo_id": "flutter",
"token_count": 8546
} | 658 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:io';
import 'dart:ui' as ui;
import 'package:flutter/foundation.dart';
import 'binding.dart';
import 'debug.dart';
import 'image_provider.dart' as image_provider;
import 'image_stream.dart';
// Method signature for _loadAsync decode callbacks.
typedef _SimpleDecoderCallback = Future<ui.Codec> Function(ui.ImmutableBuffer buffer);
/// The dart:io implementation of [image_provider.NetworkImage].
@immutable
class NetworkImage extends image_provider.ImageProvider<image_provider.NetworkImage> implements image_provider.NetworkImage {
/// Creates an object that fetches the image at the given URL.
const NetworkImage(this.url, { this.scale = 1.0, this.headers });
@override
final String url;
@override
final double scale;
@override
final Map<String, String>? headers;
@override
Future<NetworkImage> obtainKey(image_provider.ImageConfiguration configuration) {
return SynchronousFuture<NetworkImage>(this);
}
@override
ImageStreamCompleter loadBuffer(image_provider.NetworkImage key, image_provider.DecoderBufferCallback decode) {
// Ownership of this controller is handed off to [_loadAsync]; it is that
// method's responsibility to close the controller's stream when the image
// has been loaded or an error is thrown.
final StreamController<ImageChunkEvent> chunkEvents = StreamController<ImageChunkEvent>();
return MultiFrameImageStreamCompleter(
codec: _loadAsync(key as NetworkImage, chunkEvents, decode: decode),
chunkEvents: chunkEvents.stream,
scale: key.scale,
debugLabel: key.url,
informationCollector: () => <DiagnosticsNode>[
DiagnosticsProperty<image_provider.ImageProvider>('Image provider', this),
DiagnosticsProperty<image_provider.NetworkImage>('Image key', key),
],
);
}
@override
ImageStreamCompleter loadImage(image_provider.NetworkImage key, image_provider.ImageDecoderCallback decode) {
// Ownership of this controller is handed off to [_loadAsync]; it is that
// method's responsibility to close the controller's stream when the image
// has been loaded or an error is thrown.
final StreamController<ImageChunkEvent> chunkEvents = StreamController<ImageChunkEvent>();
return MultiFrameImageStreamCompleter(
codec: _loadAsync(key as NetworkImage, chunkEvents, decode: decode),
chunkEvents: chunkEvents.stream,
scale: key.scale,
debugLabel: key.url,
informationCollector: () => <DiagnosticsNode>[
DiagnosticsProperty<image_provider.ImageProvider>('Image provider', this),
DiagnosticsProperty<image_provider.NetworkImage>('Image key', key),
],
);
}
// Do not access this field directly; use [_httpClient] instead.
// We set `autoUncompress` to false to ensure that we can trust the value of
// the `Content-Length` HTTP header. We automatically uncompress the content
// in our call to [consolidateHttpClientResponseBytes].
static final HttpClient _sharedHttpClient = HttpClient()..autoUncompress = false;
static HttpClient get _httpClient {
HttpClient? client;
assert(() {
if (debugNetworkImageHttpClientProvider != null) {
client = debugNetworkImageHttpClientProvider!();
}
return true;
}());
return client ?? _sharedHttpClient;
}
Future<ui.Codec> _loadAsync(
NetworkImage key,
StreamController<ImageChunkEvent> chunkEvents, {
required _SimpleDecoderCallback decode,
}) async {
try {
assert(key == this);
final Uri resolved = Uri.base.resolve(key.url);
final HttpClientRequest request = await _httpClient.getUrl(resolved);
headers?.forEach((String name, String value) {
request.headers.add(name, value);
});
final HttpClientResponse response = await request.close();
if (response.statusCode != HttpStatus.ok) {
// The network may be only temporarily unavailable, or the file will be
// added on the server later. Avoid having future calls to resolve
// fail to check the network again.
await response.drain<List<int>>(<int>[]);
throw image_provider.NetworkImageLoadException(statusCode: response.statusCode, uri: resolved);
}
final Uint8List bytes = await consolidateHttpClientResponseBytes(
response,
onBytesReceived: (int cumulative, int? total) {
chunkEvents.add(ImageChunkEvent(
cumulativeBytesLoaded: cumulative,
expectedTotalBytes: total,
));
},
);
if (bytes.lengthInBytes == 0) {
throw Exception('NetworkImage is an empty file: $resolved');
}
return decode(await ui.ImmutableBuffer.fromUint8List(bytes));
} catch (e) {
// Depending on where the exception was thrown, the image cache may not
// have had a chance to track the key in the cache at all.
// Schedule a microtask to give the cache a chance to add the key.
scheduleMicrotask(() {
PaintingBinding.instance.imageCache.evict(key);
});
rethrow;
} finally {
chunkEvents.close();
}
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is NetworkImage
&& other.url == url
&& other.scale == scale;
}
@override
int get hashCode => Object.hash(url, scale);
@override
String toString() => '${objectRuntimeType(this, 'NetworkImage')}("$url", scale: ${scale.toStringAsFixed(1)})';
}
| flutter/packages/flutter/lib/src/painting/_network_image_io.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/_network_image_io.dart",
"repo_id": "flutter",
"token_count": 1920
} | 659 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io';
import 'dart:ui' show Image, Picture, Size;
import 'package:flutter/foundation.dart';
/// Whether to replace all shadows with solid color blocks.
///
/// This is useful when writing golden file tests (see [matchesGoldenFile]) since
/// the rendering of shadows is not guaranteed to be pixel-for-pixel identical from
/// version to version (or even from run to run).
///
/// In those tests, this is usually set to false at the beginning of a test and back
/// to true before the end of the test case.
///
/// If it remains true when the test ends, an exception is thrown to avoid state
/// leaking from one test case to another.
bool debugDisableShadows = false;
/// Signature for a method that returns an [HttpClient].
///
/// Used by [debugNetworkImageHttpClientProvider].
typedef HttpClientProvider = HttpClient Function();
/// Provider from which [NetworkImage] will get its [HttpClient] in debug builds.
///
/// If this value is unset, [NetworkImage] will use its own internally-managed
/// [HttpClient].
///
/// This setting can be overridden for testing to ensure that each test receives
/// a mock client that hasn't been affected by other tests.
///
/// This value is ignored in non-debug builds.
HttpClientProvider? debugNetworkImageHttpClientProvider;
/// Called when the framework is about to paint an [Image] to a [Canvas] with an
/// [ImageSizeInfo] that contains the decoded size of the image as well as its
/// output size.
///
/// See: [debugOnPaintImage].
typedef PaintImageCallback = void Function(ImageSizeInfo info);
/// Tracks the bytes used by a [dart:ui.Image] compared to the bytes needed to
/// paint that image without scaling it.
@immutable
class ImageSizeInfo {
/// Creates an object to track the backing size of a [dart:ui.Image] compared
/// to its display size on a [Canvas].
///
/// This class is used by the framework when it paints an image to a canvas
/// to report to `dart:developer`'s [postEvent], as well as to the
/// [debugOnPaintImage] callback if it is set.
const ImageSizeInfo({this.source, required this.displaySize, required this.imageSize});
/// A unique identifier for this image, for example its asset path or network
/// URL.
final String? source;
/// The size of the area the image will be rendered in.
final Size displaySize;
/// The size the image has been decoded to.
final Size imageSize;
/// The number of bytes needed to render the image without scaling it.
int get displaySizeInBytes => _sizeToBytes(displaySize);
/// The number of bytes used by the image in memory.
int get decodedSizeInBytes => _sizeToBytes(imageSize);
int _sizeToBytes(Size size) {
// Assume 4 bytes per pixel and that mipmapping will be used, which adds
// 4/3.
return (size.width * size.height * 4 * (4/3)).toInt();
}
/// Returns a JSON encodable representation of this object.
Map<String, Object?> toJson() {
return <String, Object?>{
'source': source,
'displaySize': <String, Object?>{
'width': displaySize.width,
'height': displaySize.height,
},
'imageSize': <String, Object?>{
'width': imageSize.width,
'height': imageSize.height,
},
'displaySizeInBytes': displaySizeInBytes,
'decodedSizeInBytes': decodedSizeInBytes,
};
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is ImageSizeInfo
&& other.source == source
&& other.imageSize == imageSize
&& other.displaySize == displaySize;
}
@override
int get hashCode => Object.hash(source, displaySize, imageSize);
@override
String toString() => 'ImageSizeInfo($source, imageSize: $imageSize, displaySize: $displaySize)';
}
/// If not null, called when the framework is about to paint an [Image] to a
/// [Canvas] with an [ImageSizeInfo] that contains the decoded size of the
/// image as well as its output size.
///
/// A test can use this callback to detect if images under test are being
/// rendered with the appropriate cache dimensions.
///
/// For example, if a 100x100 image is decoded it takes roughly 53kb in memory
/// (including mipmapping overhead). If it is only ever displayed at 50x50, it
/// would take only 13kb if the cacheHeight/cacheWidth parameters had been
/// specified at that size. This problem becomes more serious for larger
/// images, such as a high resolution image from a 12MP camera, which would be
/// 64mb when decoded.
///
/// When using this callback, developers should consider whether the image will
/// be panned or scaled up in the application, how many images are being
/// displayed, and whether the application will run on multiple devices with
/// different resolutions and memory capacities. For example, it should be fine
/// to have an image that animates from thumbnail size to full screen be at
/// a higher resolution while animating, but it would be problematic to have
/// a grid or list of such thumbnails all be at the full resolution at the same
/// time.
PaintImageCallback? debugOnPaintImage;
/// If true, the framework will color invert and horizontally flip images that
/// have been decoded to a size taking at least [debugImageOverheadAllowance]
/// bytes more than necessary.
///
/// It will also call [FlutterError.reportError] with information about the
/// image's decoded size and its display size, which can be used resize the
/// asset before shipping it, apply `cacheHeight` or `cacheWidth` parameters, or
/// directly use a [ResizeImage]. Whenever possible, resizing the image asset
/// itself should be preferred, to avoid unnecessary network traffic, disk space
/// usage, and other memory overhead incurred during decoding.
///
/// Developers using this flag should test their application on appropriate
/// devices and display sizes for their expected deployment targets when using
/// these parameters. For example, an application that responsively resizes
/// images for a desktop and mobile layout should avoid decoding all images at
/// sizes appropriate for mobile when on desktop. Applications should also avoid
/// animating these parameters, as each change will result in a newly decoded
/// image. For example, an image that always grows into view should decode only
/// at its largest size, whereas an image that normally is a thumbnail and then
/// pops into view should be decoded at its smallest size for the thumbnail and
/// the largest size when needed.
///
/// This has no effect unless asserts are enabled.
bool debugInvertOversizedImages = false;
const int _imageOverheadAllowanceDefault = 128 * 1024;
/// The number of bytes an image must use before it triggers inversion when
/// [debugInvertOversizedImages] is true.
///
/// Default is 128kb.
int debugImageOverheadAllowance = _imageOverheadAllowanceDefault;
/// Returns true if none of the painting library debug variables have been changed.
///
/// This function is used by the test framework to ensure that debug variables
/// haven't been inadvertently changed.
///
/// See [the painting library](painting/painting-library.html) for a complete
/// list.
///
/// The `debugDisableShadowsOverride` argument can be provided to override
/// the expected value for [debugDisableShadows]. (This exists because the
/// test framework itself overrides this value in some cases.)
bool debugAssertAllPaintingVarsUnset(String reason, { bool debugDisableShadowsOverride = false }) {
assert(() {
if (debugDisableShadows != debugDisableShadowsOverride ||
debugNetworkImageHttpClientProvider != null ||
debugOnPaintImage != null ||
debugInvertOversizedImages ||
debugImageOverheadAllowance != _imageOverheadAllowanceDefault) {
throw FlutterError(reason);
}
return true;
}());
return true;
}
/// The signature of [debugCaptureShaderWarmUpPicture].
///
/// Used by tests to run assertions on the [Picture] created by
/// [ShaderWarmUp.execute]. The return value indicates whether the assertions
/// pass or not.
typedef ShaderWarmUpPictureCallback = bool Function(Picture picture);
/// The signature of [debugCaptureShaderWarmUpImage].
///
/// Used by tests to run assertions on the [Image] created by
/// [ShaderWarmUp.execute]. The return value indicates whether the assertions
/// pass or not.
typedef ShaderWarmUpImageCallback = bool Function(Image image);
/// Called by [ShaderWarmUp.execute] immediately after it creates a [Picture].
///
/// Tests may use this to capture the picture and run assertions on it.
ShaderWarmUpPictureCallback debugCaptureShaderWarmUpPicture = _defaultPictureCapture;
bool _defaultPictureCapture(Picture picture) => true;
/// Called by [ShaderWarmUp.execute] immediately after it creates an [Image].
///
/// Tests may use this to capture the picture and run assertions on it.
ShaderWarmUpImageCallback debugCaptureShaderWarmUpImage = _defaultImageCapture;
bool _defaultImageCapture(Image image) => true;
| flutter/packages/flutter/lib/src/painting/debug.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/debug.dart",
"repo_id": "flutter",
"token_count": 2463
} | 660 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'basic_types.dart';
import 'borders.dart';
/// A shape with a notch in its outline.
///
/// Typically used as the outline of a 'host' widget to make a notch that
/// accommodates a 'guest' widget. e.g the [BottomAppBar] may have a notch to
/// accommodate the [FloatingActionButton].
///
/// See also:
///
/// * [ShapeBorder], which defines a shaped border without a dynamic notch.
/// * [AutomaticNotchedShape], an adapter from [ShapeBorder] to [NotchedShape].
abstract class NotchedShape {
/// Abstract const constructor. This constructor enables subclasses to provide
/// const constructors so that they can be used in const expressions.
const NotchedShape();
/// Creates a [Path] that describes the outline of the shape.
///
/// The `host` is the bounding rectangle of the shape.
///
/// The `guest` is the bounding rectangle of the shape for which a notch will
/// be made. It is null when there is no guest.
Path getOuterPath(Rect host, Rect? guest);
}
/// A rectangle with a smooth circular notch.
///
/// See also:
///
/// * [CircleBorder], a [ShapeBorder] that describes a circle.
class CircularNotchedRectangle extends NotchedShape {
/// Creates a [CircularNotchedRectangle].
///
/// The same object can be used to create multiple shapes.
const CircularNotchedRectangle();
/// Creates a [Path] that describes a rectangle with a smooth circular notch.
///
/// `host` is the bounding box for the returned shape. Conceptually this is
/// the rectangle to which the notch will be applied.
///
/// `guest` is the bounding box of a circle that the notch accommodates. All
/// points in the circle bounded by `guest` will be outside of the returned
/// path.
///
/// The notch is curve that smoothly connects the host's top edge and
/// the guest circle.
// TODO(amirh): add an example diagram here.
@override
Path getOuterPath(Rect host, Rect? guest) {
if (guest == null || !host.overlaps(guest)) {
return Path()..addRect(host);
}
// The guest's shape is a circle bounded by the guest rectangle.
// So the guest's radius is half the guest width.
final double notchRadius = guest.width / 2.0;
// We build a path for the notch from 3 segments:
// Segment A - a Bezier curve from the host's top edge to segment B.
// Segment B - an arc with radius notchRadius.
// Segment C - a Bezier curve from segment B back to the host's top edge.
//
// A detailed explanation and the derivation of the formulas below is
// available at: https://goo.gl/Ufzrqn
const double s1 = 15.0;
const double s2 = 1.0;
final double r = notchRadius;
final double a = -1.0 * r - s2;
final double b = host.top - guest.center.dy;
final double n2 = math.sqrt(b * b * r * r * (a * a + b * b - r * r));
final double p2xA = ((a * r * r) - n2) / (a * a + b * b);
final double p2xB = ((a * r * r) + n2) / (a * a + b * b);
final double p2yA = math.sqrt(r * r - p2xA * p2xA);
final double p2yB = math.sqrt(r * r - p2xB * p2xB);
final List<Offset?> p = List<Offset?>.filled(6, null);
// p0, p1, and p2 are the control points for segment A.
p[0] = Offset(a - s1, b);
p[1] = Offset(a, b);
final double cmp = b < 0 ? -1.0 : 1.0;
p[2] = cmp * p2yA > cmp * p2yB ? Offset(p2xA, p2yA) : Offset(p2xB, p2yB);
// p3, p4, and p5 are the control points for segment B, which is a mirror
// of segment A around the y axis.
p[3] = Offset(-1.0 * p[2]!.dx, p[2]!.dy);
p[4] = Offset(-1.0 * p[1]!.dx, p[1]!.dy);
p[5] = Offset(-1.0 * p[0]!.dx, p[0]!.dy);
// translate all points back to the absolute coordinate system.
for (int i = 0; i < p.length; i += 1) {
p[i] = p[i]! + guest.center;
}
return Path()
..moveTo(host.left, host.top)
..lineTo(p[0]!.dx, p[0]!.dy)
..quadraticBezierTo(p[1]!.dx, p[1]!.dy, p[2]!.dx, p[2]!.dy)
..arcToPoint(
p[3]!,
radius: Radius.circular(notchRadius),
clockwise: false,
)
..quadraticBezierTo(p[4]!.dx, p[4]!.dy, p[5]!.dx, p[5]!.dy)
..lineTo(host.right, host.top)
..lineTo(host.right, host.bottom)
..lineTo(host.left, host.bottom)
..close();
}
}
/// A [NotchedShape] created from [ShapeBorder]s.
///
/// Two shapes can be provided. The [host] is the shape of the widget that
/// uses the [NotchedShape] (typically a [BottomAppBar]). The [guest] is
/// subtracted from the [host] to create the notch (typically to make room
/// for a [FloatingActionButton]).
class AutomaticNotchedShape extends NotchedShape {
/// Creates a [NotchedShape] that is defined by two [ShapeBorder]s.
///
/// The [guest] may be null, in which case no notch is created even
/// if a guest rectangle is provided to [getOuterPath].
const AutomaticNotchedShape(this.host, [ this.guest ]);
/// The shape of the widget that uses the [NotchedShape] (typically a
/// [BottomAppBar]).
///
/// This shape cannot depend on the [TextDirection], as no text direction
/// is available to [NotchedShape]s.
final ShapeBorder host;
/// The shape to subtract from the [host] to make the notch.
///
/// This shape cannot depend on the [TextDirection], as no text direction
/// is available to [NotchedShape]s.
///
/// If this is null, [getOuterPath] ignores the guest rectangle.
final ShapeBorder? guest;
@override
Path getOuterPath(Rect hostRect, Rect? guestRect) { // ignore: avoid_renaming_method_parameters
// The parameters of this method are renamed over the baseclass because they
// would clash with properties of this object, and the use of all four of
// them in the code below is really confusing if they have the same names.
final Path hostPath = host.getOuterPath(hostRect);
if (guest != null && guestRect != null) {
final Path guestPath = guest!.getOuterPath(guestRect);
return Path.combine(PathOperation.difference, hostPath, guestPath);
}
return hostPath;
}
}
| flutter/packages/flutter/lib/src/painting/notched_shapes.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/painting/notched_shapes.dart",
"repo_id": "flutter",
"token_count": 2174
} | 661 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'simulation.dart';
// Examples can assume:
// late AnimationController _controller;
/// A simulation that applies a constant accelerating force.
///
/// Models a particle that follows Newton's second law of motion. The simulation
/// ends when the position exceeds a defined threshold.
///
/// {@tool snippet}
///
/// This method triggers an [AnimationController] (a previously constructed
/// `_controller` field) to simulate a fall of 300 pixels.
///
/// ```dart
/// void _startFall() {
/// _controller.animateWith(GravitySimulation(
/// 10.0, // acceleration, pixels per second per second
/// 0.0, // starting position, pixels
/// 300.0, // ending position, pixels
/// 0.0, // starting velocity, pixels per second
/// ));
/// }
/// ```
/// {@end-tool}
///
/// This [AnimationController] could be used with an [AnimatedBuilder] to
/// animate the position of a child as if it was falling.
///
/// The end distance threshold (the constructor's third argument) must be
/// specified as a positive number but can be reached in either the positive or
/// negative direction. For example (assuming negative numbers represent higher
/// physical positions than positive numbers, as is the case with the normal
/// [Canvas] coordinate system), if the acceleration is positive ("down") the
/// starting velocity is negative ("up"), and the starting distance is zero, the
/// particle will climb from the origin, reach a plateau, then fall back towards
/// and past the origin. If the end distance threshold is less than the height
/// of the plateau, then the simulation will end during the climb; otherwise, it
/// will end during the fall, after the particle travels below the origin by
/// that distance.
///
/// See also:
///
/// * [Curves.bounceOut], a [Curve] that has a similar aesthetics but includes
/// a bouncing effect.
class GravitySimulation extends Simulation {
/// Creates a [GravitySimulation] using the given arguments, which are,
/// respectively: an acceleration that is to be applied continually over time;
/// an initial position relative to an origin; the magnitude of the distance
/// from that origin beyond which (in either direction) to consider the
/// simulation to be "done", which must be positive; and an initial velocity.
///
/// The initial position and maximum distance are measured in arbitrary length
/// units L from an arbitrary origin. The units will match those used for [x].
///
/// The time unit T used for the arguments to [x], [dx], and [isDone],
/// combined with the aforementioned length unit, together determine the units
/// that must be used for the velocity and acceleration arguments: L/T and
/// L/T² respectively. The same units of velocity are used for the velocity
/// obtained from [dx].
GravitySimulation(
double acceleration,
double distance,
double endDistance,
double velocity,
) : assert(endDistance >= 0),
_a = acceleration,
_x = distance,
_v = velocity,
_end = endDistance;
final double _x;
final double _v;
final double _a;
final double _end;
@override
double x(double time) => _x + _v * time + 0.5 * _a * time * time;
@override
double dx(double time) => _v + time * _a;
@override
bool isDone(double time) => x(time).abs() >= _end;
@override
String toString() => '${objectRuntimeType(this, 'GravitySimulation')}(g: ${_a.toStringAsFixed(1)}, x₀: ${_x.toStringAsFixed(1)}, dx₀: ${_v.toStringAsFixed(1)}, xₘₐₓ: ±${_end.toStringAsFixed(1)})';
}
| flutter/packages/flutter/lib/src/physics/gravity_simulation.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/physics/gravity_simulation.dart",
"repo_id": "flutter",
"token_count": 1042
} | 662 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui show Color;
import 'package:flutter/foundation.dart';
import 'package:vector_math/vector_math_64.dart';
import 'box.dart';
import 'layer.dart';
import 'object.dart';
/// A context in which a [FlowDelegate] paints.
///
/// Provides information about the current size of the container and the
/// children and a mechanism for painting children.
///
/// See also:
///
/// * [FlowDelegate]
/// * [Flow]
/// * [RenderFlow]
abstract class FlowPaintingContext {
/// The size of the container in which the children can be painted.
Size get size;
/// The number of children available to paint.
int get childCount;
/// The size of the [i]th child.
///
/// If [i] is negative or exceeds [childCount], returns null.
Size? getChildSize(int i);
/// Paint the [i]th child using the given transform.
///
/// The child will be painted in a coordinate system that concatenates the
/// container's coordinate system with the given transform. The origin of the
/// parent's coordinate system is the upper left corner of the parent, with
/// x increasing rightward and y increasing downward.
///
/// The container will clip the children to its bounds.
void paintChild(int i, { Matrix4 transform, double opacity = 1.0 });
}
/// A delegate that controls the appearance of a flow layout.
///
/// Flow layouts are optimized for moving children around the screen using
/// transformation matrices. For optimal performance, construct the
/// [FlowDelegate] with an [Animation] that ticks whenever the delegate wishes
/// to change the transformation matrices for the children and avoid rebuilding
/// the [Flow] widget itself every animation frame.
///
/// See also:
///
/// * [Flow]
/// * [RenderFlow]
abstract class FlowDelegate {
/// The flow will repaint whenever [repaint] notifies its listeners.
const FlowDelegate({ Listenable? repaint }) : _repaint = repaint;
final Listenable? _repaint;
/// Override to control the size of the container for the children.
///
/// By default, the flow will be as large as possible. If this function
/// returns a size that does not respect the given constraints, the size will
/// be adjusted to be as close to the returned size as possible while still
/// respecting the constraints.
///
/// If this function depends on information other than the given constraints,
/// override [shouldRelayout] to indicate when the container should
/// relayout.
Size getSize(BoxConstraints constraints) => constraints.biggest;
/// Override to control the layout constraints given to each child.
///
/// By default, the children will receive the given constraints, which are the
/// constraints used to size the container. The children need
/// not respect the given constraints, but they are required to respect the
/// returned constraints. For example, the incoming constraints might require
/// the container to have a width of exactly 100.0 and a height of exactly
/// 100.0, but this function might give the children looser constraints that
/// let them be larger or smaller than 100.0 by 100.0.
///
/// If this function depends on information other than the given constraints,
/// override [shouldRelayout] to indicate when the container should
/// relayout.
BoxConstraints getConstraintsForChild(int i, BoxConstraints constraints) => constraints;
/// Override to paint the children of the flow.
///
/// Children can be painted in any order, but each child can be painted at
/// most once. Although the container clips the children to its own bounds, it
/// is more efficient to skip painting a child altogether rather than having
/// it paint entirely outside the container's clip.
///
/// To paint a child, call [FlowPaintingContext.paintChild] on the given
/// [FlowPaintingContext] (the `context` argument). The given context is valid
/// only within the scope of this function call and contains information (such
/// as the size of the container) that is useful for picking transformation
/// matrices for the children.
///
/// If this function depends on information other than the given context,
/// override [shouldRepaint] to indicate when the container should
/// relayout.
void paintChildren(FlowPaintingContext context);
/// Override this method to return true when the children need to be laid out.
/// This should compare the fields of the current delegate and the given
/// oldDelegate and return true if the fields are such that the layout would
/// be different.
bool shouldRelayout(covariant FlowDelegate oldDelegate) => false;
/// Override this method to return true when the children need to be
/// repainted. This should compare the fields of the current delegate and the
/// given oldDelegate and return true if the fields are such that
/// paintChildren would act differently.
///
/// The delegate can also trigger a repaint if the delegate provides the
/// repaint animation argument to this object's constructor and that animation
/// ticks. Triggering a repaint using this animation-based mechanism is more
/// efficient than rebuilding the [Flow] widget to change its delegate.
///
/// The flow container might repaint even if this function returns false, for
/// example if layout triggers painting (e.g., if [shouldRelayout] returns
/// true).
bool shouldRepaint(covariant FlowDelegate oldDelegate);
/// Override this method to include additional information in the
/// debugging data printed by [debugDumpRenderTree] and friends.
///
/// By default, returns the [runtimeType] of the class.
@override
String toString() => objectRuntimeType(this, 'FlowDelegate');
}
/// Parent data for use with [RenderFlow].
///
/// The [offset] property is ignored by [RenderFlow] and is always set to
/// [Offset.zero]. Children of a [RenderFlow] are positioned using a
/// transformation matrix, which is private to the [RenderFlow]. To set the
/// matrix, use the [FlowPaintingContext.paintChild] function from an override
/// of the [FlowDelegate.paintChildren] function.
class FlowParentData extends ContainerBoxParentData<RenderBox> {
Matrix4? _transform;
}
/// Implements the flow layout algorithm.
///
/// Flow layouts are optimized for repositioning children using transformation
/// matrices.
///
/// The flow container is sized independently from the children by the
/// [FlowDelegate.getSize] function of the delegate. The children are then sized
/// independently given the constraints from the
/// [FlowDelegate.getConstraintsForChild] function.
///
/// Rather than positioning the children during layout, the children are
/// positioned using transformation matrices during the paint phase using the
/// matrices from the [FlowDelegate.paintChildren] function. The children are thus
/// repositioned efficiently by repainting the flow, skipping layout.
///
/// The most efficient way to trigger a repaint of the flow is to supply a
/// repaint argument to the constructor of the [FlowDelegate]. The flow will
/// listen to this animation and repaint whenever the animation ticks, avoiding
/// both the build and layout phases of the pipeline.
///
/// See also:
///
/// * [FlowDelegate]
/// * [RenderStack]
class RenderFlow extends RenderBox
with ContainerRenderObjectMixin<RenderBox, FlowParentData>,
RenderBoxContainerDefaultsMixin<RenderBox, FlowParentData>
implements FlowPaintingContext {
/// Creates a render object for a flow layout.
///
/// For optimal performance, consider using children that return true from
/// [isRepaintBoundary].
RenderFlow({
List<RenderBox>? children,
required FlowDelegate delegate,
Clip clipBehavior = Clip.hardEdge,
}) : _delegate = delegate,
_clipBehavior = clipBehavior {
addAll(children);
}
@override
void setupParentData(RenderBox child) {
final ParentData? childParentData = child.parentData;
if (childParentData is FlowParentData) {
childParentData._transform = null;
} else {
child.parentData = FlowParentData();
}
}
/// The delegate that controls the transformation matrices of the children.
FlowDelegate get delegate => _delegate;
FlowDelegate _delegate;
/// When the delegate is changed to a new delegate with the same runtimeType
/// as the old delegate, this object will call the delegate's
/// [FlowDelegate.shouldRelayout] and [FlowDelegate.shouldRepaint] functions
/// to determine whether the new delegate requires this object to update its
/// layout or painting.
set delegate(FlowDelegate newDelegate) {
if (_delegate == newDelegate) {
return;
}
final FlowDelegate oldDelegate = _delegate;
_delegate = newDelegate;
if (newDelegate.runtimeType != oldDelegate.runtimeType || newDelegate.shouldRelayout(oldDelegate)) {
markNeedsLayout();
} else if (newDelegate.shouldRepaint(oldDelegate)) {
markNeedsPaint();
}
if (attached) {
oldDelegate._repaint?.removeListener(markNeedsPaint);
newDelegate._repaint?.addListener(markNeedsPaint);
}
}
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.hardEdge].
Clip get clipBehavior => _clipBehavior;
Clip _clipBehavior = Clip.hardEdge;
set clipBehavior(Clip value) {
if (value != _clipBehavior) {
_clipBehavior = value;
markNeedsPaint();
markNeedsSemanticsUpdate();
}
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
_delegate._repaint?.addListener(markNeedsPaint);
}
@override
void detach() {
_delegate._repaint?.removeListener(markNeedsPaint);
super.detach();
}
Size _getSize(BoxConstraints constraints) {
assert(constraints.debugAssertIsValid());
return constraints.constrain(_delegate.getSize(constraints));
}
@override
bool get isRepaintBoundary => true;
// TODO(ianh): It's a bit dubious to be using the getSize function from the delegate to
// figure out the intrinsic dimensions. We really should either not support intrinsics,
// or we should expose intrinsic delegate callbacks and throw if they're not implemented.
@override
double computeMinIntrinsicWidth(double height) {
final double width = _getSize(BoxConstraints.tightForFinite(height: height)).width;
if (width.isFinite) {
return width;
}
return 0.0;
}
@override
double computeMaxIntrinsicWidth(double height) {
final double width = _getSize(BoxConstraints.tightForFinite(height: height)).width;
if (width.isFinite) {
return width;
}
return 0.0;
}
@override
double computeMinIntrinsicHeight(double width) {
final double height = _getSize(BoxConstraints.tightForFinite(width: width)).height;
if (height.isFinite) {
return height;
}
return 0.0;
}
@override
double computeMaxIntrinsicHeight(double width) {
final double height = _getSize(BoxConstraints.tightForFinite(width: width)).height;
if (height.isFinite) {
return height;
}
return 0.0;
}
@override
@protected
Size computeDryLayout(covariant BoxConstraints constraints) {
return _getSize(constraints);
}
@override
void performLayout() {
final BoxConstraints constraints = this.constraints;
size = _getSize(constraints);
int i = 0;
_randomAccessChildren.clear();
RenderBox? child = firstChild;
while (child != null) {
_randomAccessChildren.add(child);
final BoxConstraints innerConstraints = _delegate.getConstraintsForChild(i, constraints);
child.layout(innerConstraints, parentUsesSize: true);
final FlowParentData childParentData = child.parentData! as FlowParentData;
childParentData.offset = Offset.zero;
child = childParentData.nextSibling;
i += 1;
}
}
// Updated during layout. Only valid if layout is not dirty.
final List<RenderBox> _randomAccessChildren = <RenderBox>[];
// Updated during paint.
final List<int> _lastPaintOrder = <int>[];
// Only valid during paint.
PaintingContext? _paintingContext;
Offset? _paintingOffset;
@override
Size? getChildSize(int i) {
if (i < 0 || i >= _randomAccessChildren.length) {
return null;
}
return _randomAccessChildren[i].size;
}
@override
void paintChild(int i, { Matrix4? transform, double opacity = 1.0 }) {
transform ??= Matrix4.identity();
final RenderBox child = _randomAccessChildren[i];
final FlowParentData childParentData = child.parentData! as FlowParentData;
assert(() {
if (childParentData._transform != null) {
throw FlutterError(
'Cannot call paintChild twice for the same child.\n'
'The flow delegate of type ${_delegate.runtimeType} attempted to '
'paint child $i multiple times, which is not permitted.',
);
}
return true;
}());
_lastPaintOrder.add(i);
childParentData._transform = transform;
// We return after assigning _transform so that the transparent child can
// still be hit tested at the correct location.
if (opacity == 0.0) {
return;
}
void painter(PaintingContext context, Offset offset) {
context.paintChild(child, offset);
}
if (opacity == 1.0) {
_paintingContext!.pushTransform(needsCompositing, _paintingOffset!, transform, painter);
} else {
_paintingContext!.pushOpacity(_paintingOffset!, ui.Color.getAlphaFromOpacity(opacity), (PaintingContext context, Offset offset) {
context.pushTransform(needsCompositing, offset, transform!, painter);
});
}
}
void _paintWithDelegate(PaintingContext context, Offset offset) {
_lastPaintOrder.clear();
_paintingContext = context;
_paintingOffset = offset;
for (final RenderBox child in _randomAccessChildren) {
final FlowParentData childParentData = child.parentData! as FlowParentData;
childParentData._transform = null;
}
try {
_delegate.paintChildren(this);
} finally {
_paintingContext = null;
_paintingOffset = null;
}
}
@override
void paint(PaintingContext context, Offset offset) {
_clipRectLayer.layer = context.pushClipRect(
needsCompositing,
offset,
Offset.zero & size,
_paintWithDelegate,
clipBehavior: clipBehavior,
oldLayer: _clipRectLayer.layer,
);
}
final LayerHandle<ClipRectLayer> _clipRectLayer = LayerHandle<ClipRectLayer>();
@override
void dispose() {
_clipRectLayer.layer = null;
super.dispose();
}
@override
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
final List<RenderBox> children = getChildrenAsList();
for (int i = _lastPaintOrder.length - 1; i >= 0; --i) {
final int childIndex = _lastPaintOrder[i];
if (childIndex >= children.length) {
continue;
}
final RenderBox child = children[childIndex];
final FlowParentData childParentData = child.parentData! as FlowParentData;
final Matrix4? transform = childParentData._transform;
if (transform == null) {
continue;
}
final bool absorbed = result.addWithPaintTransform(
transform: transform,
position: position,
hitTest: (BoxHitTestResult result, Offset position) {
return child.hitTest(result, position: position);
},
);
if (absorbed) {
return true;
}
}
return false;
}
@override
void applyPaintTransform(RenderBox child, Matrix4 transform) {
final FlowParentData childParentData = child.parentData! as FlowParentData;
if (childParentData._transform != null) {
transform.multiply(childParentData._transform!);
}
super.applyPaintTransform(child, transform);
}
}
| flutter/packages/flutter/lib/src/rendering/flow.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/flow.dart",
"repo_id": "flutter",
"token_count": 4866
} | 663 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/foundation.dart';
import 'box.dart';
import 'debug.dart';
import 'debug_overflow_indicator.dart';
import 'layer.dart';
import 'object.dart';
import 'stack.dart' show RelativeRect;
/// Signature for a function that transforms a [BoxConstraints] to another
/// [BoxConstraints].
///
/// Used by [RenderConstraintsTransformBox] and [ConstraintsTransformBox].
/// Typically the caller requires the returned [BoxConstraints] to be
/// [BoxConstraints.isNormalized].
typedef BoxConstraintsTransform = BoxConstraints Function(BoxConstraints constraints);
/// Abstract class for one-child-layout render boxes that provide control over
/// the child's position.
abstract class RenderShiftedBox extends RenderBox with RenderObjectWithChildMixin<RenderBox> {
/// Initializes the [child] property for subclasses.
RenderShiftedBox(RenderBox? child) {
this.child = child;
}
@override
double computeMinIntrinsicWidth(double height) {
return child?.getMinIntrinsicWidth(height) ?? 0.0;
}
@override
double computeMaxIntrinsicWidth(double height) {
return child?.getMaxIntrinsicWidth(height) ?? 0.0;
}
@override
double computeMinIntrinsicHeight(double width) {
return child?.getMinIntrinsicHeight(width) ?? 0.0;
}
@override
double computeMaxIntrinsicHeight(double width) {
return child?.getMaxIntrinsicHeight(width) ?? 0.0;
}
@override
double? computeDistanceToActualBaseline(TextBaseline baseline) {
double? result;
final RenderBox? child = this.child;
if (child != null) {
assert(!debugNeedsLayout);
result = child.getDistanceToActualBaseline(baseline);
final BoxParentData childParentData = child.parentData! as BoxParentData;
if (result != null) {
result += childParentData.offset.dy;
}
} else {
result = super.computeDistanceToActualBaseline(baseline);
}
return result;
}
@override
void paint(PaintingContext context, Offset offset) {
final RenderBox? child = this.child;
if (child != null) {
final BoxParentData childParentData = child.parentData! as BoxParentData;
context.paintChild(child, childParentData.offset + offset);
}
}
@override
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
final RenderBox? child = this.child;
if (child != null) {
final BoxParentData childParentData = child.parentData! as BoxParentData;
return result.addWithPaintOffset(
offset: childParentData.offset,
position: position,
hitTest: (BoxHitTestResult result, Offset transformed) {
assert(transformed == position - childParentData.offset);
return child.hitTest(result, position: transformed);
},
);
}
return false;
}
}
/// Insets its child by the given padding.
///
/// When passing layout constraints to its child, padding shrinks the
/// constraints by the given padding, causing the child to layout at a smaller
/// size. Padding then sizes itself to its child's size, inflated by the
/// padding, effectively creating empty space around the child.
class RenderPadding extends RenderShiftedBox {
/// Creates a render object that insets its child.
///
/// The [padding] argument must have non-negative insets.
RenderPadding({
required EdgeInsetsGeometry padding,
TextDirection? textDirection,
RenderBox? child,
}) : assert(padding.isNonNegative),
_textDirection = textDirection,
_padding = padding,
super(child);
EdgeInsets? _resolvedPadding;
void _resolve() {
if (_resolvedPadding != null) {
return;
}
_resolvedPadding = padding.resolve(textDirection);
assert(_resolvedPadding!.isNonNegative);
}
void _markNeedResolution() {
_resolvedPadding = null;
markNeedsLayout();
}
/// The amount to pad the child in each dimension.
///
/// If this is set to an [EdgeInsetsDirectional] object, then [textDirection]
/// must not be null.
EdgeInsetsGeometry get padding => _padding;
EdgeInsetsGeometry _padding;
set padding(EdgeInsetsGeometry value) {
assert(value.isNonNegative);
if (_padding == value) {
return;
}
_padding = value;
_markNeedResolution();
}
/// The text direction with which to resolve [padding].
///
/// This may be changed to null, but only after the [padding] has been changed
/// to a value that does not depend on the direction.
TextDirection? get textDirection => _textDirection;
TextDirection? _textDirection;
set textDirection(TextDirection? value) {
if (_textDirection == value) {
return;
}
_textDirection = value;
_markNeedResolution();
}
@override
double computeMinIntrinsicWidth(double height) {
_resolve();
final double totalHorizontalPadding = _resolvedPadding!.left + _resolvedPadding!.right;
final double totalVerticalPadding = _resolvedPadding!.top + _resolvedPadding!.bottom;
if (child != null) {
// Relies on double.infinity absorption.
return child!.getMinIntrinsicWidth(math.max(0.0, height - totalVerticalPadding)) + totalHorizontalPadding;
}
return totalHorizontalPadding;
}
@override
double computeMaxIntrinsicWidth(double height) {
_resolve();
final double totalHorizontalPadding = _resolvedPadding!.left + _resolvedPadding!.right;
final double totalVerticalPadding = _resolvedPadding!.top + _resolvedPadding!.bottom;
if (child != null) {
// Relies on double.infinity absorption.
return child!.getMaxIntrinsicWidth(math.max(0.0, height - totalVerticalPadding)) + totalHorizontalPadding;
}
return totalHorizontalPadding;
}
@override
double computeMinIntrinsicHeight(double width) {
_resolve();
final double totalHorizontalPadding = _resolvedPadding!.left + _resolvedPadding!.right;
final double totalVerticalPadding = _resolvedPadding!.top + _resolvedPadding!.bottom;
if (child != null) {
// Relies on double.infinity absorption.
return child!.getMinIntrinsicHeight(math.max(0.0, width - totalHorizontalPadding)) + totalVerticalPadding;
}
return totalVerticalPadding;
}
@override
double computeMaxIntrinsicHeight(double width) {
_resolve();
final double totalHorizontalPadding = _resolvedPadding!.left + _resolvedPadding!.right;
final double totalVerticalPadding = _resolvedPadding!.top + _resolvedPadding!.bottom;
if (child != null) {
// Relies on double.infinity absorption.
return child!.getMaxIntrinsicHeight(math.max(0.0, width - totalHorizontalPadding)) + totalVerticalPadding;
}
return totalVerticalPadding;
}
@override
@protected
Size computeDryLayout(covariant BoxConstraints constraints) {
_resolve();
assert(_resolvedPadding != null);
if (child == null) {
return constraints.constrain(Size(
_resolvedPadding!.left + _resolvedPadding!.right,
_resolvedPadding!.top + _resolvedPadding!.bottom,
));
}
final BoxConstraints innerConstraints = constraints.deflate(_resolvedPadding!);
final Size childSize = child!.getDryLayout(innerConstraints);
return constraints.constrain(Size(
_resolvedPadding!.left + childSize.width + _resolvedPadding!.right,
_resolvedPadding!.top + childSize.height + _resolvedPadding!.bottom,
));
}
@override
void performLayout() {
final BoxConstraints constraints = this.constraints;
_resolve();
assert(_resolvedPadding != null);
if (child == null) {
size = constraints.constrain(Size(
_resolvedPadding!.left + _resolvedPadding!.right,
_resolvedPadding!.top + _resolvedPadding!.bottom,
));
return;
}
final BoxConstraints innerConstraints = constraints.deflate(_resolvedPadding!);
child!.layout(innerConstraints, parentUsesSize: true);
final BoxParentData childParentData = child!.parentData! as BoxParentData;
childParentData.offset = Offset(_resolvedPadding!.left, _resolvedPadding!.top);
size = constraints.constrain(Size(
_resolvedPadding!.left + child!.size.width + _resolvedPadding!.right,
_resolvedPadding!.top + child!.size.height + _resolvedPadding!.bottom,
));
}
@override
void debugPaintSize(PaintingContext context, Offset offset) {
super.debugPaintSize(context, offset);
assert(() {
final Rect outerRect = offset & size;
debugPaintPadding(context.canvas, outerRect, child != null ? _resolvedPadding!.deflateRect(outerRect) : null);
return true;
}());
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('padding', padding));
properties.add(EnumProperty<TextDirection>('textDirection', textDirection, defaultValue: null));
}
}
/// Abstract class for one-child-layout render boxes that use a
/// [AlignmentGeometry] to align their children.
abstract class RenderAligningShiftedBox extends RenderShiftedBox {
/// Initializes member variables for subclasses.
///
/// The [textDirection] must be non-null if the [alignment] is
/// direction-sensitive.
RenderAligningShiftedBox({
AlignmentGeometry alignment = Alignment.center,
required TextDirection? textDirection,
RenderBox? child,
}) : _alignment = alignment,
_textDirection = textDirection,
super(child);
Alignment? _resolvedAlignment;
void _resolve() {
if (_resolvedAlignment != null) {
return;
}
_resolvedAlignment = alignment.resolve(textDirection);
}
void _markNeedResolution() {
_resolvedAlignment = null;
markNeedsLayout();
}
/// How to align the child.
///
/// The x and y values of the alignment control the horizontal and vertical
/// alignment, respectively. An x value of -1.0 means that the left edge of
/// the child is aligned with the left edge of the parent whereas an x value
/// of 1.0 means that the right edge of the child is aligned with the right
/// edge of the parent. Other values interpolate (and extrapolate) linearly.
/// For example, a value of 0.0 means that the center of the child is aligned
/// with the center of the parent.
///
/// If this is set to an [AlignmentDirectional] object, then
/// [textDirection] must not be null.
AlignmentGeometry get alignment => _alignment;
AlignmentGeometry _alignment;
/// Sets the alignment to a new value, and triggers a layout update.
set alignment(AlignmentGeometry value) {
if (_alignment == value) {
return;
}
_alignment = value;
_markNeedResolution();
}
/// The text direction with which to resolve [alignment].
///
/// This may be changed to null, but only after [alignment] has been changed
/// to a value that does not depend on the direction.
TextDirection? get textDirection => _textDirection;
TextDirection? _textDirection;
set textDirection(TextDirection? value) {
if (_textDirection == value) {
return;
}
_textDirection = value;
_markNeedResolution();
}
/// Apply the current [alignment] to the [child].
///
/// Subclasses should call this method if they have a child, to have
/// this class perform the actual alignment. If there is no child,
/// do not call this method.
///
/// This method must be called after the child has been laid out and
/// this object's own size has been set.
@protected
void alignChild() {
_resolve();
assert(child != null);
assert(!child!.debugNeedsLayout);
assert(child!.hasSize);
assert(hasSize);
assert(_resolvedAlignment != null);
final BoxParentData childParentData = child!.parentData! as BoxParentData;
childParentData.offset = _resolvedAlignment!.alongOffset(size - child!.size as Offset);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<AlignmentGeometry>('alignment', alignment));
properties.add(EnumProperty<TextDirection>('textDirection', textDirection, defaultValue: null));
}
}
/// Positions its child using an [AlignmentGeometry].
///
/// For example, to align a box at the bottom right, you would pass this box a
/// tight constraint that is bigger than the child's natural size,
/// with an alignment of [Alignment.bottomRight].
///
/// By default, sizes to be as big as possible in both axes. If either axis is
/// unconstrained, then in that direction it will be sized to fit the child's
/// dimensions. Using widthFactor and heightFactor you can force this latter
/// behavior in all cases.
class RenderPositionedBox extends RenderAligningShiftedBox {
/// Creates a render object that positions its child.
RenderPositionedBox({
super.child,
double? widthFactor,
double? heightFactor,
super.alignment,
super.textDirection,
}) : assert(widthFactor == null || widthFactor >= 0.0),
assert(heightFactor == null || heightFactor >= 0.0),
_widthFactor = widthFactor,
_heightFactor = heightFactor;
/// If non-null, sets its width to the child's width multiplied by this factor.
///
/// Can be both greater and less than 1.0 but must be positive.
double? get widthFactor => _widthFactor;
double? _widthFactor;
set widthFactor(double? value) {
assert(value == null || value >= 0.0);
if (_widthFactor == value) {
return;
}
_widthFactor = value;
markNeedsLayout();
}
/// If non-null, sets its height to the child's height multiplied by this factor.
///
/// Can be both greater and less than 1.0 but must be positive.
double? get heightFactor => _heightFactor;
double? _heightFactor;
set heightFactor(double? value) {
assert(value == null || value >= 0.0);
if (_heightFactor == value) {
return;
}
_heightFactor = value;
markNeedsLayout();
}
@override
double computeMinIntrinsicWidth(double height) {
return super.computeMinIntrinsicWidth(height) * (_widthFactor ?? 1);
}
@override
double computeMaxIntrinsicWidth(double height) {
return super.computeMaxIntrinsicWidth(height) * (_widthFactor ?? 1);
}
@override
double computeMinIntrinsicHeight(double width) {
return super.computeMinIntrinsicHeight(width) * (_heightFactor ?? 1);
}
@override
double computeMaxIntrinsicHeight(double width) {
return super.computeMaxIntrinsicHeight(width) * (_heightFactor ?? 1);
}
@override
@protected
Size computeDryLayout(covariant BoxConstraints constraints) {
final bool shrinkWrapWidth = _widthFactor != null || constraints.maxWidth == double.infinity;
final bool shrinkWrapHeight = _heightFactor != null || constraints.maxHeight == double.infinity;
if (child != null) {
final Size childSize = child!.getDryLayout(constraints.loosen());
return constraints.constrain(Size(
shrinkWrapWidth ? childSize.width * (_widthFactor ?? 1.0) : double.infinity,
shrinkWrapHeight ? childSize.height * (_heightFactor ?? 1.0) : double.infinity,
));
}
return constraints.constrain(Size(
shrinkWrapWidth ? 0.0 : double.infinity,
shrinkWrapHeight ? 0.0 : double.infinity,
));
}
@override
void performLayout() {
final BoxConstraints constraints = this.constraints;
final bool shrinkWrapWidth = _widthFactor != null || constraints.maxWidth == double.infinity;
final bool shrinkWrapHeight = _heightFactor != null || constraints.maxHeight == double.infinity;
if (child != null) {
child!.layout(constraints.loosen(), parentUsesSize: true);
size = constraints.constrain(Size(
shrinkWrapWidth ? child!.size.width * (_widthFactor ?? 1.0) : double.infinity,
shrinkWrapHeight ? child!.size.height * (_heightFactor ?? 1.0) : double.infinity,
));
alignChild();
} else {
size = constraints.constrain(Size(
shrinkWrapWidth ? 0.0 : double.infinity,
shrinkWrapHeight ? 0.0 : double.infinity,
));
}
}
@override
void debugPaintSize(PaintingContext context, Offset offset) {
super.debugPaintSize(context, offset);
assert(() {
final Paint paint;
if (child != null && !child!.size.isEmpty) {
final Path path;
paint = Paint()
..style = PaintingStyle.stroke
..strokeWidth = 1.0
..color = const Color(0xFFFFFF00);
path = Path();
final BoxParentData childParentData = child!.parentData! as BoxParentData;
if (childParentData.offset.dy > 0.0) {
// vertical alignment arrows
final double headSize = math.min(childParentData.offset.dy * 0.2, 10.0);
path
..moveTo(offset.dx + size.width / 2.0, offset.dy)
..relativeLineTo(0.0, childParentData.offset.dy - headSize)
..relativeLineTo(headSize, 0.0)
..relativeLineTo(-headSize, headSize)
..relativeLineTo(-headSize, -headSize)
..relativeLineTo(headSize, 0.0)
..moveTo(offset.dx + size.width / 2.0, offset.dy + size.height)
..relativeLineTo(0.0, -childParentData.offset.dy + headSize)
..relativeLineTo(headSize, 0.0)
..relativeLineTo(-headSize, -headSize)
..relativeLineTo(-headSize, headSize)
..relativeLineTo(headSize, 0.0);
context.canvas.drawPath(path, paint);
}
if (childParentData.offset.dx > 0.0) {
// horizontal alignment arrows
final double headSize = math.min(childParentData.offset.dx * 0.2, 10.0);
path
..moveTo(offset.dx, offset.dy + size.height / 2.0)
..relativeLineTo(childParentData.offset.dx - headSize, 0.0)
..relativeLineTo(0.0, headSize)
..relativeLineTo(headSize, -headSize)
..relativeLineTo(-headSize, -headSize)
..relativeLineTo(0.0, headSize)
..moveTo(offset.dx + size.width, offset.dy + size.height / 2.0)
..relativeLineTo(-childParentData.offset.dx + headSize, 0.0)
..relativeLineTo(0.0, headSize)
..relativeLineTo(-headSize, -headSize)
..relativeLineTo(headSize, -headSize)
..relativeLineTo(0.0, headSize);
context.canvas.drawPath(path, paint);
}
} else {
paint = Paint()
..color = const Color(0x90909090);
context.canvas.drawRect(offset & size, paint);
}
return true;
}());
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('widthFactor', _widthFactor, ifNull: 'expand'));
properties.add(DoubleProperty('heightFactor', _heightFactor, ifNull: 'expand'));
}
}
/// How much space should be occupied by the [OverflowBox] if there is no
/// overflow.
enum OverflowBoxFit {
/// The widget will size itself to be as large as the parent allows.
max,
/// The widget will follow the child's size.
///
/// More specifically, the render object will size itself to match the size of
/// its child within the constraints of its parent, or as small as the
/// parent allows if no child is set.
deferToChild,
}
/// A render object that imposes different constraints on its child than it gets
/// from its parent, possibly allowing the child to overflow the parent.
///
/// A render overflow box proxies most functions in the render box protocol to
/// its child, except that when laying out its child, it passes constraints
/// based on the minWidth, maxWidth, minHeight, and maxHeight fields instead of
/// just passing the parent's constraints in. Specifically, it overrides any of
/// the equivalent fields on the constraints given by the parent with the
/// constraints given by these fields for each such field that is not null. It
/// then sizes itself based on the parent's constraints' maxWidth and maxHeight,
/// ignoring the child's dimensions.
///
/// For example, if you wanted a box to always render 50 pixels high, regardless
/// of where it was rendered, you would wrap it in a
/// RenderConstrainedOverflowBox with minHeight and maxHeight set to 50.0.
/// Generally speaking, to avoid confusing behavior around hit testing, a
/// RenderConstrainedOverflowBox should usually be wrapped in a RenderClipRect.
///
/// The child is positioned according to [alignment]. To position a smaller
/// child inside a larger parent, use [RenderPositionedBox] and
/// [RenderConstrainedBox] rather than RenderConstrainedOverflowBox.
///
/// See also:
///
/// * [RenderConstraintsTransformBox] for a render object that applies an
/// arbitrary transform to its constraints before sizing its child using
/// the new constraints, treating any overflow as error.
/// * [RenderSizedOverflowBox], a render object that is a specific size but
/// passes its original constraints through to its child, which it allows to
/// overflow.
class RenderConstrainedOverflowBox extends RenderAligningShiftedBox {
/// Creates a render object that lets its child overflow itself.
RenderConstrainedOverflowBox({
super.child,
double? minWidth,
double? maxWidth,
double? minHeight,
double? maxHeight,
OverflowBoxFit fit = OverflowBoxFit.max,
super.alignment,
super.textDirection,
}) : _minWidth = minWidth,
_maxWidth = maxWidth,
_minHeight = minHeight,
_maxHeight = maxHeight,
_fit = fit;
/// The minimum width constraint to give the child. Set this to null (the
/// default) to use the constraint from the parent instead.
double? get minWidth => _minWidth;
double? _minWidth;
set minWidth(double? value) {
if (_minWidth == value) {
return;
}
_minWidth = value;
markNeedsLayout();
}
/// The maximum width constraint to give the child. Set this to null (the
/// default) to use the constraint from the parent instead.
double? get maxWidth => _maxWidth;
double? _maxWidth;
set maxWidth(double? value) {
if (_maxWidth == value) {
return;
}
_maxWidth = value;
markNeedsLayout();
}
/// The minimum height constraint to give the child. Set this to null (the
/// default) to use the constraint from the parent instead.
double? get minHeight => _minHeight;
double? _minHeight;
set minHeight(double? value) {
if (_minHeight == value) {
return;
}
_minHeight = value;
markNeedsLayout();
}
/// The maximum height constraint to give the child. Set this to null (the
/// default) to use the constraint from the parent instead.
double? get maxHeight => _maxHeight;
double? _maxHeight;
set maxHeight(double? value) {
if (_maxHeight == value) {
return;
}
_maxHeight = value;
markNeedsLayout();
}
/// The way to size the render object.
///
/// This only affects scenario when the child does not indeed overflow.
/// If set to [OverflowBoxFit.deferToChild], the render object will size
/// itself to match the size of its child within the constraints of its
/// parent, or as small as the parent allows if no child is set.
/// If set to [OverflowBoxFit.max] (the default), the
/// render object will size itself to be as large as the parent allows.
OverflowBoxFit get fit => _fit;
OverflowBoxFit _fit;
set fit(OverflowBoxFit value) {
if (_fit == value) {
return;
}
_fit = value;
markNeedsLayoutForSizedByParentChange();
}
BoxConstraints _getInnerConstraints(BoxConstraints constraints) {
return BoxConstraints(
minWidth: _minWidth ?? constraints.minWidth,
maxWidth: _maxWidth ?? constraints.maxWidth,
minHeight: _minHeight ?? constraints.minHeight,
maxHeight: _maxHeight ?? constraints.maxHeight,
);
}
@override
bool get sizedByParent {
return switch (fit) {
OverflowBoxFit.max => true,
// If deferToChild, the size will be as small as its child when non-overflowing,
// thus it cannot be sizedByParent.
OverflowBoxFit.deferToChild => false,
};
}
@override
@protected
Size computeDryLayout(covariant BoxConstraints constraints) {
return switch (fit) {
OverflowBoxFit.max => constraints.biggest,
OverflowBoxFit.deferToChild => child?.getDryLayout(constraints) ?? constraints.smallest,
};
}
@override
void performLayout() {
if (child != null) {
child!.layout(_getInnerConstraints(constraints), parentUsesSize: true);
switch (fit) {
case OverflowBoxFit.max:
assert(sizedByParent);
case OverflowBoxFit.deferToChild:
size = constraints.constrain(child!.size);
}
alignChild();
} else {
switch (fit) {
case OverflowBoxFit.max:
assert(sizedByParent);
case OverflowBoxFit.deferToChild:
size = constraints.smallest;
}
}
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('minWidth', minWidth, ifNull: 'use parent minWidth constraint'));
properties.add(DoubleProperty('maxWidth', maxWidth, ifNull: 'use parent maxWidth constraint'));
properties.add(DoubleProperty('minHeight', minHeight, ifNull: 'use parent minHeight constraint'));
properties.add(DoubleProperty('maxHeight', maxHeight, ifNull: 'use parent maxHeight constraint'));
properties.add(EnumProperty<OverflowBoxFit>('fit', fit));
}
}
/// A [RenderBox] that applies an arbitrary transform to its constraints,
/// and sizes its child using the resulting [BoxConstraints], optionally
/// clipping, or treating the overflow as an error.
///
/// This [RenderBox] sizes its child using a [BoxConstraints] created by
/// applying [constraintsTransform] to this [RenderBox]'s own [constraints].
/// This box will then attempt to adopt the same size, within the limits of its
/// own constraints. If it ends up with a different size, it will align the
/// child based on [alignment]. If the box cannot expand enough to accommodate
/// the entire child, the child will be clipped if [clipBehavior] is not
/// [Clip.none].
///
/// In debug mode, if [clipBehavior] is [Clip.none] and the child overflows the
/// container, a warning will be printed on the console, and black and yellow
/// striped areas will appear where the overflow occurs.
///
/// When [child] is null, this [RenderBox] takes the smallest possible size and
/// never overflows.
///
/// This [RenderBox] can be used to ensure some of [child]'s natural dimensions
/// are honored, and get an early warning during development otherwise. For
/// instance, if [child] requires a minimum height to fully display its content,
/// [constraintsTransform] can be set to a function that removes the `maxHeight`
/// constraint from the incoming [BoxConstraints], so that if the parent
/// [RenderObject] fails to provide enough vertical space, a warning will be
/// displayed in debug mode, while still allowing [child] to grow vertically.
///
/// See also:
///
/// * [ConstraintsTransformBox], the widget that makes use of this
/// [RenderObject] and exposes the same functionality.
/// * [RenderConstrainedBox], which renders a box which imposes constraints
/// on its child.
/// * [RenderConstrainedOverflowBox], which renders a box that imposes different
/// constraints on its child than it gets from its parent, possibly allowing
/// the child to overflow the parent.
/// * [RenderConstraintsTransformBox] for a render object that applies an
/// arbitrary transform to its constraints before sizing its child using
/// the new constraints, treating any overflow as error.
class RenderConstraintsTransformBox extends RenderAligningShiftedBox with DebugOverflowIndicatorMixin {
/// Creates a [RenderBox] that sizes itself to the child and modifies the
/// [constraints] before passing it down to that child.
RenderConstraintsTransformBox({
required super.alignment,
required super.textDirection,
required BoxConstraintsTransform constraintsTransform,
super.child,
Clip clipBehavior = Clip.none,
}) : _constraintsTransform = constraintsTransform,
_clipBehavior = clipBehavior;
/// {@macro flutter.widgets.constraintsTransform}
BoxConstraintsTransform get constraintsTransform => _constraintsTransform;
BoxConstraintsTransform _constraintsTransform;
set constraintsTransform(BoxConstraintsTransform value) {
if (_constraintsTransform == value) {
return;
}
_constraintsTransform = value;
// The RenderObject only needs layout if the new transform maps the current
// `constraints` to a different value, or the render object has never been
// laid out before.
final bool needsLayout = _childConstraints == null
|| _childConstraints != value(constraints);
if (needsLayout) {
markNeedsLayout();
}
}
/// {@macro flutter.material.Material.clipBehavior}
///
/// {@macro flutter.widgets.ConstraintsTransformBox.clipBehavior}
///
/// Defaults to [Clip.none].
Clip get clipBehavior => _clipBehavior;
Clip _clipBehavior;
set clipBehavior(Clip value) {
if (value != _clipBehavior) {
_clipBehavior = value;
markNeedsPaint();
markNeedsSemanticsUpdate();
}
}
@override
double computeMinIntrinsicHeight(double width) {
return super.computeMinIntrinsicHeight(
constraintsTransform(BoxConstraints(maxWidth: width)).maxWidth,
);
}
@override
double computeMaxIntrinsicHeight(double width) {
return super.computeMaxIntrinsicHeight(
constraintsTransform(BoxConstraints(maxWidth: width)).maxWidth,
);
}
@override
double computeMinIntrinsicWidth(double height) {
return super.computeMinIntrinsicWidth(
constraintsTransform(BoxConstraints(maxHeight: height)).maxHeight,
);
}
@override
double computeMaxIntrinsicWidth(double height) {
return super.computeMaxIntrinsicWidth(
constraintsTransform(BoxConstraints(maxHeight: height)).maxHeight,
);
}
@override
@protected
Size computeDryLayout(covariant BoxConstraints constraints) {
final Size? childSize = child?.getDryLayout(constraintsTransform(constraints));
return childSize == null ? constraints.smallest : constraints.constrain(childSize);
}
Rect _overflowContainerRect = Rect.zero;
Rect _overflowChildRect = Rect.zero;
bool _isOverflowing = false;
BoxConstraints? _childConstraints;
@override
void performLayout() {
final BoxConstraints constraints = this.constraints;
final RenderBox? child = this.child;
if (child != null) {
final BoxConstraints childConstraints = constraintsTransform(constraints);
assert(childConstraints.isNormalized, '$childConstraints is not normalized');
_childConstraints = childConstraints;
child.layout(childConstraints, parentUsesSize: true);
size = constraints.constrain(child.size);
alignChild();
final BoxParentData childParentData = child.parentData! as BoxParentData;
_overflowContainerRect = Offset.zero & size;
_overflowChildRect = childParentData.offset & child.size;
} else {
size = constraints.smallest;
_overflowContainerRect = Rect.zero;
_overflowChildRect = Rect.zero;
}
_isOverflowing = RelativeRect.fromRect(_overflowContainerRect, _overflowChildRect).hasInsets;
}
@override
void paint(PaintingContext context, Offset offset) {
// There's no point in drawing the child if we're empty, or there is no
// child.
if (child == null || size.isEmpty) {
return;
}
if (!_isOverflowing) {
super.paint(context, offset);
return;
}
// We have overflow and the clipBehavior isn't none. Clip it.
_clipRectLayer.layer = context.pushClipRect(
needsCompositing,
offset,
Offset.zero & size,
super.paint,
clipBehavior: clipBehavior,
oldLayer: _clipRectLayer.layer,
);
// Display the overflow indicator if clipBehavior is Clip.none.
assert(() {
switch (clipBehavior) {
case Clip.none:
paintOverflowIndicator(context, offset, _overflowContainerRect, _overflowChildRect);
case Clip.hardEdge:
case Clip.antiAlias:
case Clip.antiAliasWithSaveLayer:
break;
}
return true;
}());
}
final LayerHandle<ClipRectLayer> _clipRectLayer = LayerHandle<ClipRectLayer>();
@override
void dispose() {
_clipRectLayer.layer = null;
super.dispose();
}
@override
Rect? describeApproximatePaintClip(RenderObject child) {
switch (clipBehavior) {
case Clip.none:
return null;
case Clip.hardEdge:
case Clip.antiAlias:
case Clip.antiAliasWithSaveLayer:
return _isOverflowing ? Offset.zero & size : null;
}
}
@override
String toStringShort() {
String header = super.toStringShort();
if (!kReleaseMode) {
if (_isOverflowing) {
header += ' OVERFLOWING';
}
}
return header;
}
}
/// A render object that is a specific size but passes its original constraints
/// through to its child, which it allows to overflow.
///
/// If the child's resulting size differs from this render object's size, then
/// the child is aligned according to the [alignment] property.
///
/// See also:
///
/// * [RenderConstraintsTransformBox] for a render object that applies an
/// arbitrary transform to its constraints before sizing its child using
/// the new constraints, treating any overflow as error.
/// * [RenderConstrainedOverflowBox] for a render object that imposes
/// different constraints on its child than it gets from its parent,
/// possibly allowing the child to overflow the parent.
class RenderSizedOverflowBox extends RenderAligningShiftedBox {
/// Creates a render box of a given size that lets its child overflow.
///
/// The [textDirection] argument must not be null if the [alignment] is
/// direction-sensitive.
RenderSizedOverflowBox({
super.child,
required Size requestedSize,
super.alignment,
super.textDirection,
}) : _requestedSize = requestedSize;
/// The size this render box should attempt to be.
Size get requestedSize => _requestedSize;
Size _requestedSize;
set requestedSize(Size value) {
if (_requestedSize == value) {
return;
}
_requestedSize = value;
markNeedsLayout();
}
@override
double computeMinIntrinsicWidth(double height) {
return _requestedSize.width;
}
@override
double computeMaxIntrinsicWidth(double height) {
return _requestedSize.width;
}
@override
double computeMinIntrinsicHeight(double width) {
return _requestedSize.height;
}
@override
double computeMaxIntrinsicHeight(double width) {
return _requestedSize.height;
}
@override
double? computeDistanceToActualBaseline(TextBaseline baseline) {
if (child != null) {
return child!.getDistanceToActualBaseline(baseline);
}
return super.computeDistanceToActualBaseline(baseline);
}
@override
@protected
Size computeDryLayout(covariant BoxConstraints constraints) {
return constraints.constrain(_requestedSize);
}
@override
void performLayout() {
size = constraints.constrain(_requestedSize);
if (child != null) {
child!.layout(constraints, parentUsesSize: true);
alignChild();
}
}
}
/// Sizes its child to a fraction of the total available space.
///
/// For both its width and height, this render object imposes a tight
/// constraint on its child that is a multiple (typically less than 1.0) of the
/// maximum constraint it received from its parent on that axis. If the factor
/// for a given axis is null, then the constraints from the parent are just
/// passed through instead.
///
/// It then tries to size itself to the size of its child. Where this is not
/// possible (e.g. if the constraints from the parent are themselves tight), the
/// child is aligned according to [alignment].
class RenderFractionallySizedOverflowBox extends RenderAligningShiftedBox {
/// Creates a render box that sizes its child to a fraction of the total available space.
///
/// If non-null, the [widthFactor] and [heightFactor] arguments must be
/// non-negative.
///
/// The [textDirection] must be non-null if the [alignment] is
/// direction-sensitive.
RenderFractionallySizedOverflowBox({
super.child,
double? widthFactor,
double? heightFactor,
super.alignment,
super.textDirection,
}) : _widthFactor = widthFactor,
_heightFactor = heightFactor {
assert(_widthFactor == null || _widthFactor! >= 0.0);
assert(_heightFactor == null || _heightFactor! >= 0.0);
}
/// If non-null, the factor of the incoming width to use.
///
/// If non-null, the child is given a tight width constraint that is the max
/// incoming width constraint multiplied by this factor. If null, the child is
/// given the incoming width constraints.
double? get widthFactor => _widthFactor;
double? _widthFactor;
set widthFactor(double? value) {
assert(value == null || value >= 0.0);
if (_widthFactor == value) {
return;
}
_widthFactor = value;
markNeedsLayout();
}
/// If non-null, the factor of the incoming height to use.
///
/// If non-null, the child is given a tight height constraint that is the max
/// incoming width constraint multiplied by this factor. If null, the child is
/// given the incoming width constraints.
double? get heightFactor => _heightFactor;
double? _heightFactor;
set heightFactor(double? value) {
assert(value == null || value >= 0.0);
if (_heightFactor == value) {
return;
}
_heightFactor = value;
markNeedsLayout();
}
BoxConstraints _getInnerConstraints(BoxConstraints constraints) {
double minWidth = constraints.minWidth;
double maxWidth = constraints.maxWidth;
if (_widthFactor != null) {
final double width = maxWidth * _widthFactor!;
minWidth = width;
maxWidth = width;
}
double minHeight = constraints.minHeight;
double maxHeight = constraints.maxHeight;
if (_heightFactor != null) {
final double height = maxHeight * _heightFactor!;
minHeight = height;
maxHeight = height;
}
return BoxConstraints(
minWidth: minWidth,
maxWidth: maxWidth,
minHeight: minHeight,
maxHeight: maxHeight,
);
}
@override
double computeMinIntrinsicWidth(double height) {
final double result;
if (child == null) {
result = super.computeMinIntrinsicWidth(height);
} else { // the following line relies on double.infinity absorption
result = child!.getMinIntrinsicWidth(height * (_heightFactor ?? 1.0));
}
assert(result.isFinite);
return result / (_widthFactor ?? 1.0);
}
@override
double computeMaxIntrinsicWidth(double height) {
final double result;
if (child == null) {
result = super.computeMaxIntrinsicWidth(height);
} else { // the following line relies on double.infinity absorption
result = child!.getMaxIntrinsicWidth(height * (_heightFactor ?? 1.0));
}
assert(result.isFinite);
return result / (_widthFactor ?? 1.0);
}
@override
double computeMinIntrinsicHeight(double width) {
final double result;
if (child == null) {
result = super.computeMinIntrinsicHeight(width);
} else { // the following line relies on double.infinity absorption
result = child!.getMinIntrinsicHeight(width * (_widthFactor ?? 1.0));
}
assert(result.isFinite);
return result / (_heightFactor ?? 1.0);
}
@override
double computeMaxIntrinsicHeight(double width) {
final double result;
if (child == null) {
result = super.computeMaxIntrinsicHeight(width);
} else { // the following line relies on double.infinity absorption
result = child!.getMaxIntrinsicHeight(width * (_widthFactor ?? 1.0));
}
assert(result.isFinite);
return result / (_heightFactor ?? 1.0);
}
@override
@protected
Size computeDryLayout(covariant BoxConstraints constraints) {
if (child != null) {
final Size childSize = child!.getDryLayout(_getInnerConstraints(constraints));
return constraints.constrain(childSize);
}
return constraints.constrain(_getInnerConstraints(constraints).constrain(Size.zero));
}
@override
void performLayout() {
if (child != null) {
child!.layout(_getInnerConstraints(constraints), parentUsesSize: true);
size = constraints.constrain(child!.size);
alignChild();
} else {
size = constraints.constrain(_getInnerConstraints(constraints).constrain(Size.zero));
}
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('widthFactor', _widthFactor, ifNull: 'pass-through'));
properties.add(DoubleProperty('heightFactor', _heightFactor, ifNull: 'pass-through'));
}
}
/// A delegate for computing the layout of a render object with a single child.
///
/// Used by [CustomSingleChildLayout] (in the widgets library) and
/// [RenderCustomSingleChildLayoutBox] (in the rendering library).
///
/// When asked to layout, [CustomSingleChildLayout] first calls [getSize] with
/// its incoming constraints to determine its size. It then calls
/// [getConstraintsForChild] to determine the constraints to apply to the child.
/// After the child completes its layout, [RenderCustomSingleChildLayoutBox]
/// calls [getPositionForChild] to determine the child's position.
///
/// The [shouldRelayout] method is called when a new instance of the class
/// is provided, to check if the new instance actually represents different
/// information.
///
/// The most efficient way to trigger a relayout is to supply a `relayout`
/// argument to the constructor of the [SingleChildLayoutDelegate]. The custom
/// layout will listen to this value and relayout whenever the Listenable
/// notifies its listeners, such as when an [Animation] ticks. This allows
/// the custom layout to avoid the build phase of the pipeline.
///
/// See also:
///
/// * [CustomSingleChildLayout], the widget that uses this delegate.
/// * [RenderCustomSingleChildLayoutBox], render object that uses this
/// delegate.
abstract class SingleChildLayoutDelegate {
/// Creates a layout delegate.
///
/// The layout will update whenever [relayout] notifies its listeners.
const SingleChildLayoutDelegate({ Listenable? relayout }) : _relayout = relayout;
final Listenable? _relayout;
/// The size of this object given the incoming constraints.
///
/// Defaults to the biggest size that satisfies the given constraints.
Size getSize(BoxConstraints constraints) => constraints.biggest;
/// The constraints for the child given the incoming constraints.
///
/// During layout, the child is given the layout constraints returned by this
/// function. The child is required to pick a size for itself that satisfies
/// these constraints.
///
/// Defaults to the given constraints.
BoxConstraints getConstraintsForChild(BoxConstraints constraints) => constraints;
/// The position where the child should be placed.
///
/// The `size` argument is the size of the parent, which might be different
/// from the value returned by [getSize] if that size doesn't satisfy the
/// constraints passed to [getSize]. The `childSize` argument is the size of
/// the child, which will satisfy the constraints returned by
/// [getConstraintsForChild].
///
/// Defaults to positioning the child in the upper left corner of the parent.
Offset getPositionForChild(Size size, Size childSize) => Offset.zero;
/// Called whenever a new instance of the custom layout delegate class is
/// provided to the [RenderCustomSingleChildLayoutBox] object, or any time
/// that a new [CustomSingleChildLayout] object is created with a new instance
/// of the custom layout delegate class (which amounts to the same thing,
/// because the latter is implemented in terms of the former).
///
/// If the new instance represents different information than the old
/// instance, then the method should return true, otherwise it should return
/// false.
///
/// If the method returns false, then the [getSize],
/// [getConstraintsForChild], and [getPositionForChild] calls might be
/// optimized away.
///
/// It's possible that the layout methods will get called even if
/// [shouldRelayout] returns false (e.g. if an ancestor changed its layout).
/// It's also possible that the layout method will get called
/// without [shouldRelayout] being called at all (e.g. if the parent changes
/// size).
bool shouldRelayout(covariant SingleChildLayoutDelegate oldDelegate);
}
/// Defers the layout of its single child to a delegate.
///
/// The delegate can determine the layout constraints for the child and can
/// decide where to position the child. The delegate can also determine the size
/// of the parent, but the size of the parent cannot depend on the size of the
/// child.
class RenderCustomSingleChildLayoutBox extends RenderShiftedBox {
/// Creates a render box that defers its layout to a delegate.
///
/// The [delegate] argument must not be null.
RenderCustomSingleChildLayoutBox({
RenderBox? child,
required SingleChildLayoutDelegate delegate,
}) : _delegate = delegate,
super(child);
/// A delegate that controls this object's layout.
SingleChildLayoutDelegate get delegate => _delegate;
SingleChildLayoutDelegate _delegate;
set delegate(SingleChildLayoutDelegate newDelegate) {
if (_delegate == newDelegate) {
return;
}
final SingleChildLayoutDelegate oldDelegate = _delegate;
if (newDelegate.runtimeType != oldDelegate.runtimeType || newDelegate.shouldRelayout(oldDelegate)) {
markNeedsLayout();
}
_delegate = newDelegate;
if (attached) {
oldDelegate._relayout?.removeListener(markNeedsLayout);
newDelegate._relayout?.addListener(markNeedsLayout);
}
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
_delegate._relayout?.addListener(markNeedsLayout);
}
@override
void detach() {
_delegate._relayout?.removeListener(markNeedsLayout);
super.detach();
}
Size _getSize(BoxConstraints constraints) {
return constraints.constrain(_delegate.getSize(constraints));
}
// TODO(ianh): It's a bit dubious to be using the getSize function from the delegate to
// figure out the intrinsic dimensions. We really should either not support intrinsics,
// or we should expose intrinsic delegate callbacks and throw if they're not implemented.
@override
double computeMinIntrinsicWidth(double height) {
final double width = _getSize(BoxConstraints.tightForFinite(height: height)).width;
if (width.isFinite) {
return width;
}
return 0.0;
}
@override
double computeMaxIntrinsicWidth(double height) {
final double width = _getSize(BoxConstraints.tightForFinite(height: height)).width;
if (width.isFinite) {
return width;
}
return 0.0;
}
@override
double computeMinIntrinsicHeight(double width) {
final double height = _getSize(BoxConstraints.tightForFinite(width: width)).height;
if (height.isFinite) {
return height;
}
return 0.0;
}
@override
double computeMaxIntrinsicHeight(double width) {
final double height = _getSize(BoxConstraints.tightForFinite(width: width)).height;
if (height.isFinite) {
return height;
}
return 0.0;
}
@override
@protected
Size computeDryLayout(covariant BoxConstraints constraints) {
return _getSize(constraints);
}
@override
void performLayout() {
size = _getSize(constraints);
if (child != null) {
final BoxConstraints childConstraints = delegate.getConstraintsForChild(constraints);
assert(childConstraints.debugAssertIsValid(isAppliedConstraint: true));
child!.layout(childConstraints, parentUsesSize: !childConstraints.isTight);
final BoxParentData childParentData = child!.parentData! as BoxParentData;
childParentData.offset = delegate.getPositionForChild(size, childConstraints.isTight ? childConstraints.smallest : child!.size);
}
}
}
/// Shifts the child down such that the child's baseline (or the
/// bottom of the child, if the child has no baseline) is [baseline]
/// logical pixels below the top of this box, then sizes this box to
/// contain the child.
///
/// If [baseline] is less than the distance from the top of the child
/// to the baseline of the child, then the child will overflow the top
/// of the box. This is typically not desirable, in particular, that
/// part of the child will not be found when doing hit tests, so the
/// user cannot interact with that part of the child.
///
/// This box will be sized so that its bottom is coincident with the
/// bottom of the child. This means if this box shifts the child down,
/// there will be space between the top of this box and the top of the
/// child, but there is never space between the bottom of the child
/// and the bottom of the box.
class RenderBaseline extends RenderShiftedBox {
/// Creates a [RenderBaseline] object.
RenderBaseline({
RenderBox? child,
required double baseline,
required TextBaseline baselineType,
}) : _baseline = baseline,
_baselineType = baselineType,
super(child);
/// The number of logical pixels from the top of this box at which to position
/// the child's baseline.
double get baseline => _baseline;
double _baseline;
set baseline(double value) {
if (_baseline == value) {
return;
}
_baseline = value;
markNeedsLayout();
}
/// The type of baseline to use for positioning the child.
TextBaseline get baselineType => _baselineType;
TextBaseline _baselineType;
set baselineType(TextBaseline value) {
if (_baselineType == value) {
return;
}
_baselineType = value;
markNeedsLayout();
}
@override
@protected
Size computeDryLayout(covariant BoxConstraints constraints) {
if (child != null) {
assert(debugCannotComputeDryLayout(
reason: 'Baseline metrics are only available after a full layout.',
));
return Size.zero;
}
return constraints.smallest;
}
@override
void performLayout() {
if (child != null) {
final BoxConstraints constraints = this.constraints;
child!.layout(constraints.loosen(), parentUsesSize: true);
final double childBaseline = child!.getDistanceToBaseline(baselineType)!;
final double actualBaseline = baseline;
final double top = actualBaseline - childBaseline;
final BoxParentData childParentData = child!.parentData! as BoxParentData;
childParentData.offset = Offset(0.0, top);
final Size childSize = child!.size;
size = constraints.constrain(Size(childSize.width, top + childSize.height));
} else {
size = constraints.smallest;
}
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('baseline', baseline));
properties.add(EnumProperty<TextBaseline>('baselineType', baselineType));
}
}
| flutter/packages/flutter/lib/src/rendering/shifted_box.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/shifted_box.dart",
"repo_id": "flutter",
"token_count": 16877
} | 664 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'package:flutter/animation.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/semantics.dart';
import 'box.dart';
import 'debug.dart';
import 'layer.dart';
import 'object.dart';
import 'sliver.dart';
import 'viewport_offset.dart';
/// The unit of measurement for a [Viewport.cacheExtent].
enum CacheExtentStyle {
/// Treat the [Viewport.cacheExtent] as logical pixels.
pixel,
/// Treat the [Viewport.cacheExtent] as a multiplier of the main axis extent.
viewport,
}
/// An interface for render objects that are bigger on the inside.
///
/// Some render objects, such as [RenderViewport], present a portion of their
/// content, which can be controlled by a [ViewportOffset]. This interface lets
/// the framework recognize such render objects and interact with them without
/// having specific knowledge of all the various types of viewports.
abstract interface class RenderAbstractViewport extends RenderObject {
/// Returns the [RenderAbstractViewport] that most tightly encloses the given
/// render object.
///
/// If the object does not have a [RenderAbstractViewport] as an ancestor,
/// this function returns null.
///
/// See also:
///
/// * [RenderAbstractViewport.of], which is similar to this method, but
/// asserts if no [RenderAbstractViewport] ancestor is found.
static RenderAbstractViewport? maybeOf(RenderObject? object) {
while (object != null) {
if (object is RenderAbstractViewport) {
return object;
}
object = object.parent;
}
return null;
}
/// Returns the [RenderAbstractViewport] that most tightly encloses the given
/// render object.
///
/// If the object does not have a [RenderAbstractViewport] as an ancestor,
/// this function will assert in debug mode, and throw an exception in release
/// mode.
///
/// See also:
///
/// * [RenderAbstractViewport.maybeOf], which is similar to this method, but
/// returns null if no [RenderAbstractViewport] ancestor is found.
static RenderAbstractViewport of(RenderObject? object) {
final RenderAbstractViewport? viewport = maybeOf(object);
assert(() {
if (viewport == null) {
throw FlutterError(
'RenderAbstractViewport.of() was called with a render object that was '
'not a descendant of a RenderAbstractViewport.\n'
'No RenderAbstractViewport render object ancestor could be found starting '
'from the object that was passed to RenderAbstractViewport.of().\n'
'The render object where the viewport search started was:\n'
' $object',
);
}
return true;
}());
return viewport!;
}
/// Returns the offset that would be needed to reveal the `target`
/// [RenderObject].
///
/// This is used by [RenderViewportBase.showInViewport], which is
/// itself used by [RenderObject.showOnScreen] for
/// [RenderViewportBase], which is in turn used by the semantics
/// system to implement scrolling for accessibility tools.
///
/// The optional `rect` parameter describes which area of that `target` object
/// should be revealed in the viewport. If `rect` is null, the entire
/// `target` [RenderObject] (as defined by its [RenderObject.paintBounds])
/// will be revealed. If `rect` is provided it has to be given in the
/// coordinate system of the `target` object.
///
/// The `alignment` argument describes where the target should be positioned
/// after applying the returned offset. If `alignment` is 0.0, the child must
/// be positioned as close to the leading edge of the viewport as possible. If
/// `alignment` is 1.0, the child must be positioned as close to the trailing
/// edge of the viewport as possible. If `alignment` is 0.5, the child must be
/// positioned as close to the center of the viewport as possible.
///
/// The `target` might not be a direct child of this viewport but it must be a
/// descendant of the viewport. Other viewports in between this viewport and
/// the `target` will not be adjusted.
///
/// This method assumes that the content of the viewport moves linearly, i.e.
/// when the offset of the viewport is changed by x then `target` also moves
/// by x within the viewport.
///
/// The optional [Axis] is used by
/// [RenderTwoDimensionalViewport.getOffsetToReveal] to
/// determine which of the two axes to compute an offset for. One dimensional
/// subclasses like [RenderViewportBase] and [RenderListWheelViewport]
/// will ignore the `axis` value if provided, since there is only one [Axis].
///
/// If the `axis` is omitted when called on [RenderTwoDimensionalViewport],
/// the [RenderTwoDimensionalViewport.mainAxis] is used. To reveal an object
/// properly in both axes, this method should be called for each [Axis] as the
/// returned [RevealedOffset.offset] only represents the offset of one of the
/// the two [ScrollPosition]s.
///
/// See also:
///
/// * [RevealedOffset], which describes the return value of this method.
RevealedOffset getOffsetToReveal(
RenderObject target,
double alignment, {
Rect? rect,
Axis? axis,
});
/// The default value for the cache extent of the viewport.
///
/// This default assumes [CacheExtentStyle.pixel].
///
/// See also:
///
/// * [RenderViewportBase.cacheExtent] for a definition of the cache extent.
static const double defaultCacheExtent = 250.0;
}
/// Return value for [RenderAbstractViewport.getOffsetToReveal].
///
/// It indicates the [offset] required to reveal an element in a viewport and
/// the [rect] position said element would have in the viewport at that
/// [offset].
class RevealedOffset {
/// Instantiates a return value for [RenderAbstractViewport.getOffsetToReveal].
const RevealedOffset({
required this.offset,
required this.rect,
});
/// Offset for the viewport to reveal a specific element in the viewport.
///
/// See also:
///
/// * [RenderAbstractViewport.getOffsetToReveal], which calculates this
/// value for a specific element.
final double offset;
/// The [Rect] in the outer coordinate system of the viewport at which the
/// to-be-revealed element would be located if the viewport's offset is set
/// to [offset].
///
/// A viewport usually has two coordinate systems and works as an adapter
/// between the two:
///
/// The inner coordinate system has its origin at the top left corner of the
/// content that moves inside the viewport. The origin of this coordinate
/// system usually moves around relative to the leading edge of the viewport
/// when the viewport offset changes.
///
/// The outer coordinate system has its origin at the top left corner of the
/// visible part of the viewport. This origin stays at the same position
/// regardless of the current viewport offset.
///
/// In other words: [rect] describes where the revealed element would be
/// located relative to the top left corner of the visible part of the
/// viewport if the viewport's offset is set to [offset].
///
/// See also:
///
/// * [RenderAbstractViewport.getOffsetToReveal], which calculates this
/// value for a specific element.
final Rect rect;
/// Determines which provided leading or trailing edge of the viewport, as
/// [RevealedOffset]s, will be used for [RenderViewportBase.showInViewport]
/// accounting for the size and already visible portion of the [RenderObject]
/// that is being revealed.
///
/// Also used by [RenderTwoDimensionalViewport.showInViewport] for each
/// horizontal and vertical [Axis].
///
/// If the target [RenderObject] is already fully visible, this will return
/// null.
static RevealedOffset? clampOffset({
required RevealedOffset leadingEdgeOffset,
required RevealedOffset trailingEdgeOffset,
required double currentOffset,
}) {
// scrollOffset
// 0 +---------+
// | |
// _ | |
// viewport position | | |
// with `descendant` at | | | _
// trailing edge |_ | xxxxxxx | | viewport position
// | | | with `descendant` at
// | | _| leading edge
// | |
// 800 +---------+
//
// `trailingEdgeOffset`: Distance from scrollOffset 0 to the start of the
// viewport on the left in image above.
// `leadingEdgeOffset`: Distance from scrollOffset 0 to the start of the
// viewport on the right in image above.
//
// The viewport position on the left is achieved by setting `offset.pixels`
// to `trailingEdgeOffset`, the one on the right by setting it to
// `leadingEdgeOffset`.
final bool inverted = leadingEdgeOffset.offset < trailingEdgeOffset.offset;
final RevealedOffset smaller;
final RevealedOffset larger;
(smaller, larger) = inverted
? (leadingEdgeOffset, trailingEdgeOffset)
: (trailingEdgeOffset, leadingEdgeOffset);
if (currentOffset > larger.offset) {
return larger;
} else if (currentOffset < smaller.offset) {
return smaller;
} else {
return null;
}
}
@override
String toString() {
return '${objectRuntimeType(this, 'RevealedOffset')}(offset: $offset, rect: $rect)';
}
}
/// A base class for render objects that are bigger on the inside.
///
/// This render object provides the shared code for render objects that host
/// [RenderSliver] render objects inside a [RenderBox]. The viewport establishes
/// an [axisDirection], which orients the sliver's coordinate system, which is
/// based on scroll offsets rather than Cartesian coordinates.
///
/// The viewport also listens to an [offset], which determines the
/// [SliverConstraints.scrollOffset] input to the sliver layout protocol.
///
/// Subclasses typically override [performLayout] and call
/// [layoutChildSequence], perhaps multiple times.
///
/// See also:
///
/// * [RenderSliver], which explains more about the Sliver protocol.
/// * [RenderBox], which explains more about the Box protocol.
/// * [RenderSliverToBoxAdapter], which allows a [RenderBox] object to be
/// placed inside a [RenderSliver] (the opposite of this class).
abstract class RenderViewportBase<ParentDataClass extends ContainerParentDataMixin<RenderSliver>>
extends RenderBox with ContainerRenderObjectMixin<RenderSliver, ParentDataClass>
implements RenderAbstractViewport {
/// Initializes fields for subclasses.
///
/// The [cacheExtent], if null, defaults to [RenderAbstractViewport.defaultCacheExtent].
///
/// The [cacheExtent] must be specified if [cacheExtentStyle] is not [CacheExtentStyle.pixel].
RenderViewportBase({
AxisDirection axisDirection = AxisDirection.down,
required AxisDirection crossAxisDirection,
required ViewportOffset offset,
double? cacheExtent,
CacheExtentStyle cacheExtentStyle = CacheExtentStyle.pixel,
Clip clipBehavior = Clip.hardEdge,
}) : assert(axisDirectionToAxis(axisDirection) != axisDirectionToAxis(crossAxisDirection)),
assert(cacheExtent != null || cacheExtentStyle == CacheExtentStyle.pixel),
_axisDirection = axisDirection,
_crossAxisDirection = crossAxisDirection,
_offset = offset,
_cacheExtent = cacheExtent ?? RenderAbstractViewport.defaultCacheExtent,
_cacheExtentStyle = cacheExtentStyle,
_clipBehavior = clipBehavior;
/// Report the semantics of this node, for example for accessibility purposes.
///
/// [RenderViewportBase] adds [RenderViewport.useTwoPaneSemantics] to the
/// provided [SemanticsConfiguration] to support children using
/// [RenderViewport.excludeFromScrolling].
///
/// This method should be overridden by subclasses that have interesting
/// semantic information. Overriding subclasses should call
/// `super.describeSemanticsConfiguration(config)` to ensure
/// [RenderViewport.useTwoPaneSemantics] is still added to `config`.
///
/// See also:
///
/// * [RenderObject.describeSemanticsConfiguration], for important
/// details about not mutating a [SemanticsConfiguration] out of context.
@override
void describeSemanticsConfiguration(SemanticsConfiguration config) {
super.describeSemanticsConfiguration(config);
config.addTagForChildren(RenderViewport.useTwoPaneSemantics);
}
@override
void visitChildrenForSemantics(RenderObjectVisitor visitor) {
childrenInPaintOrder
.where((RenderSliver sliver) => sliver.geometry!.visible || sliver.geometry!.cacheExtent > 0.0)
.forEach(visitor);
}
/// The direction in which the [SliverConstraints.scrollOffset] increases.
///
/// For example, if the [axisDirection] is [AxisDirection.down], a scroll
/// offset of zero is at the top of the viewport and increases towards the
/// bottom of the viewport.
AxisDirection get axisDirection => _axisDirection;
AxisDirection _axisDirection;
set axisDirection(AxisDirection value) {
if (value == _axisDirection) {
return;
}
_axisDirection = value;
markNeedsLayout();
}
/// The direction in which child should be laid out in the cross axis.
///
/// For example, if the [axisDirection] is [AxisDirection.down], this property
/// is typically [AxisDirection.left] if the ambient [TextDirection] is
/// [TextDirection.rtl] and [AxisDirection.right] if the ambient
/// [TextDirection] is [TextDirection.ltr].
AxisDirection get crossAxisDirection => _crossAxisDirection;
AxisDirection _crossAxisDirection;
set crossAxisDirection(AxisDirection value) {
if (value == _crossAxisDirection) {
return;
}
_crossAxisDirection = value;
markNeedsLayout();
}
/// The axis along which the viewport scrolls.
///
/// For example, if the [axisDirection] is [AxisDirection.down], then the
/// [axis] is [Axis.vertical] and the viewport scrolls vertically.
Axis get axis => axisDirectionToAxis(axisDirection);
/// Which part of the content inside the viewport should be visible.
///
/// The [ViewportOffset.pixels] value determines the scroll offset that the
/// viewport uses to select which part of its content to display. As the user
/// scrolls the viewport, this value changes, which changes the content that
/// is displayed.
ViewportOffset get offset => _offset;
ViewportOffset _offset;
set offset(ViewportOffset value) {
if (value == _offset) {
return;
}
if (attached) {
_offset.removeListener(markNeedsLayout);
}
_offset = value;
if (attached) {
_offset.addListener(markNeedsLayout);
}
// We need to go through layout even if the new offset has the same pixels
// value as the old offset so that we will apply our viewport and content
// dimensions.
markNeedsLayout();
}
// TODO(ianh): cacheExtent/cacheExtentStyle should be a single
// object that specifies both the scalar value and the unit, not a
// pair of independent setters. Changing that would allow a more
// rational API and would let us make the getter non-nullable.
/// {@template flutter.rendering.RenderViewportBase.cacheExtent}
/// The viewport has an area before and after the visible area to cache items
/// that are about to become visible when the user scrolls.
///
/// Items that fall in this cache area are laid out even though they are not
/// (yet) visible on screen. The [cacheExtent] describes how many pixels
/// the cache area extends before the leading edge and after the trailing edge
/// of the viewport.
///
/// The total extent, which the viewport will try to cover with children, is
/// [cacheExtent] before the leading edge + extent of the main axis +
/// [cacheExtent] after the trailing edge.
///
/// The cache area is also used to implement implicit accessibility scrolling
/// on iOS: When the accessibility focus moves from an item in the visible
/// viewport to an invisible item in the cache area, the framework will bring
/// that item into view with an (implicit) scroll action.
/// {@endtemplate}
///
/// The getter can never return null, but the field is nullable
/// because the setter can be set to null to reset the value to
/// [RenderAbstractViewport.defaultCacheExtent] (in which case
/// [cacheExtentStyle] must be [CacheExtentStyle.pixel]).
///
/// See also:
///
/// * [cacheExtentStyle], which controls the units of the [cacheExtent].
double? get cacheExtent => _cacheExtent;
double _cacheExtent;
set cacheExtent(double? value) {
value ??= RenderAbstractViewport.defaultCacheExtent;
if (value == _cacheExtent) {
return;
}
_cacheExtent = value;
markNeedsLayout();
}
/// This value is set during layout based on the [CacheExtentStyle].
///
/// When the style is [CacheExtentStyle.viewport], it is the main axis extent
/// of the viewport multiplied by the requested cache extent, which is still
/// expressed in pixels.
double? _calculatedCacheExtent;
/// {@template flutter.rendering.RenderViewportBase.cacheExtentStyle}
/// Controls how the [cacheExtent] is interpreted.
///
/// If set to [CacheExtentStyle.pixel], the [cacheExtent] will be
/// treated as a logical pixels, and the default [cacheExtent] is
/// [RenderAbstractViewport.defaultCacheExtent].
///
/// If set to [CacheExtentStyle.viewport], the [cacheExtent] will be
/// treated as a multiplier for the main axis extent of the
/// viewport. In this case there is no default [cacheExtent]; it
/// must be explicitly specified.
/// {@endtemplate}
///
/// Changing the [cacheExtentStyle] without also changing the [cacheExtent]
/// is rarely the correct choice.
CacheExtentStyle get cacheExtentStyle => _cacheExtentStyle;
CacheExtentStyle _cacheExtentStyle;
set cacheExtentStyle(CacheExtentStyle value) {
if (value == _cacheExtentStyle) {
return;
}
_cacheExtentStyle = value;
markNeedsLayout();
}
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.hardEdge].
Clip get clipBehavior => _clipBehavior;
Clip _clipBehavior = Clip.hardEdge;
set clipBehavior(Clip value) {
if (value != _clipBehavior) {
_clipBehavior = value;
markNeedsPaint();
markNeedsSemanticsUpdate();
}
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
_offset.addListener(markNeedsLayout);
}
@override
void detach() {
_offset.removeListener(markNeedsLayout);
super.detach();
}
/// Throws an exception saying that the object does not support returning
/// intrinsic dimensions if, in debug mode, we are not in the
/// [RenderObject.debugCheckingIntrinsics] mode.
///
/// This is used by [computeMinIntrinsicWidth] et al because viewports do not
/// generally support returning intrinsic dimensions. See the discussion at
/// [computeMinIntrinsicWidth].
@protected
bool debugThrowIfNotCheckingIntrinsics() {
assert(() {
if (!RenderObject.debugCheckingIntrinsics) {
assert(this is! RenderShrinkWrappingViewport); // it has its own message
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('$runtimeType does not support returning intrinsic dimensions.'),
ErrorDescription(
'Calculating the intrinsic dimensions would require instantiating every child of '
'the viewport, which defeats the point of viewports being lazy.',
),
ErrorHint(
'If you are merely trying to shrink-wrap the viewport in the main axis direction, '
'consider a RenderShrinkWrappingViewport render object (ShrinkWrappingViewport widget), '
'which achieves that effect without implementing the intrinsic dimension API.',
),
]);
}
return true;
}());
return true;
}
@override
double computeMinIntrinsicWidth(double height) {
assert(debugThrowIfNotCheckingIntrinsics());
return 0.0;
}
@override
double computeMaxIntrinsicWidth(double height) {
assert(debugThrowIfNotCheckingIntrinsics());
return 0.0;
}
@override
double computeMinIntrinsicHeight(double width) {
assert(debugThrowIfNotCheckingIntrinsics());
return 0.0;
}
@override
double computeMaxIntrinsicHeight(double width) {
assert(debugThrowIfNotCheckingIntrinsics());
return 0.0;
}
@override
bool get isRepaintBoundary => true;
/// Determines the size and position of some of the children of the viewport.
///
/// This function is the workhorse of `performLayout` implementations in
/// subclasses.
///
/// Layout starts with `child`, proceeds according to the `advance` callback,
/// and stops once `advance` returns null.
///
/// * `scrollOffset` is the [SliverConstraints.scrollOffset] to pass the
/// first child. The scroll offset is adjusted by
/// [SliverGeometry.scrollExtent] for subsequent children.
/// * `overlap` is the [SliverConstraints.overlap] to pass the first child.
/// The overlay is adjusted by the [SliverGeometry.paintOrigin] and
/// [SliverGeometry.paintExtent] for subsequent children.
/// * `layoutOffset` is the layout offset at which to place the first child.
/// The layout offset is updated by the [SliverGeometry.layoutExtent] for
/// subsequent children.
/// * `remainingPaintExtent` is [SliverConstraints.remainingPaintExtent] to
/// pass the first child. The remaining paint extent is updated by the
/// [SliverGeometry.layoutExtent] for subsequent children.
/// * `mainAxisExtent` is the [SliverConstraints.viewportMainAxisExtent] to
/// pass to each child.
/// * `crossAxisExtent` is the [SliverConstraints.crossAxisExtent] to pass to
/// each child.
/// * `growthDirection` is the [SliverConstraints.growthDirection] to pass to
/// each child.
///
/// Returns the first non-zero [SliverGeometry.scrollOffsetCorrection]
/// encountered, if any. Otherwise returns 0.0. Typical callers will call this
/// function repeatedly until it returns 0.0.
@protected
double layoutChildSequence({
required RenderSliver? child,
required double scrollOffset,
required double overlap,
required double layoutOffset,
required double remainingPaintExtent,
required double mainAxisExtent,
required double crossAxisExtent,
required GrowthDirection growthDirection,
required RenderSliver? Function(RenderSliver child) advance,
required double remainingCacheExtent,
required double cacheOrigin,
}) {
assert(scrollOffset.isFinite);
assert(scrollOffset >= 0.0);
final double initialLayoutOffset = layoutOffset;
final ScrollDirection adjustedUserScrollDirection =
applyGrowthDirectionToScrollDirection(offset.userScrollDirection, growthDirection);
double maxPaintOffset = layoutOffset + overlap;
double precedingScrollExtent = 0.0;
while (child != null) {
final double sliverScrollOffset = scrollOffset <= 0.0 ? 0.0 : scrollOffset;
// If the scrollOffset is too small we adjust the paddedOrigin because it
// doesn't make sense to ask a sliver for content before its scroll
// offset.
final double correctedCacheOrigin = math.max(cacheOrigin, -sliverScrollOffset);
final double cacheExtentCorrection = cacheOrigin - correctedCacheOrigin;
assert(sliverScrollOffset >= correctedCacheOrigin.abs());
assert(correctedCacheOrigin <= 0.0);
assert(sliverScrollOffset >= 0.0);
assert(cacheExtentCorrection <= 0.0);
child.layout(SliverConstraints(
axisDirection: axisDirection,
growthDirection: growthDirection,
userScrollDirection: adjustedUserScrollDirection,
scrollOffset: sliverScrollOffset,
precedingScrollExtent: precedingScrollExtent,
overlap: maxPaintOffset - layoutOffset,
remainingPaintExtent: math.max(0.0, remainingPaintExtent - layoutOffset + initialLayoutOffset),
crossAxisExtent: crossAxisExtent,
crossAxisDirection: crossAxisDirection,
viewportMainAxisExtent: mainAxisExtent,
remainingCacheExtent: math.max(0.0, remainingCacheExtent + cacheExtentCorrection),
cacheOrigin: correctedCacheOrigin,
), parentUsesSize: true);
final SliverGeometry childLayoutGeometry = child.geometry!;
assert(childLayoutGeometry.debugAssertIsValid());
// If there is a correction to apply, we'll have to start over.
if (childLayoutGeometry.scrollOffsetCorrection != null) {
return childLayoutGeometry.scrollOffsetCorrection!;
}
// We use the child's paint origin in our coordinate system as the
// layoutOffset we store in the child's parent data.
final double effectiveLayoutOffset = layoutOffset + childLayoutGeometry.paintOrigin;
// `effectiveLayoutOffset` becomes meaningless once we moved past the trailing edge
// because `childLayoutGeometry.layoutExtent` is zero. Using the still increasing
// 'scrollOffset` to roughly position these invisible slivers in the right order.
if (childLayoutGeometry.visible || scrollOffset > 0) {
updateChildLayoutOffset(child, effectiveLayoutOffset, growthDirection);
} else {
updateChildLayoutOffset(child, -scrollOffset + initialLayoutOffset, growthDirection);
}
maxPaintOffset = math.max(effectiveLayoutOffset + childLayoutGeometry.paintExtent, maxPaintOffset);
scrollOffset -= childLayoutGeometry.scrollExtent;
precedingScrollExtent += childLayoutGeometry.scrollExtent;
layoutOffset += childLayoutGeometry.layoutExtent;
if (childLayoutGeometry.cacheExtent != 0.0) {
remainingCacheExtent -= childLayoutGeometry.cacheExtent - cacheExtentCorrection;
cacheOrigin = math.min(correctedCacheOrigin + childLayoutGeometry.cacheExtent, 0.0);
}
updateOutOfBandData(growthDirection, childLayoutGeometry);
// move on to the next child
child = advance(child);
}
// we made it without a correction, whee!
return 0.0;
}
@override
Rect? describeApproximatePaintClip(RenderSliver child) {
switch (clipBehavior) {
case Clip.none:
return null;
case Clip.hardEdge:
case Clip.antiAlias:
case Clip.antiAliasWithSaveLayer:
break;
}
final Rect viewportClip = Offset.zero & size;
// The child's viewportMainAxisExtent can be infinite when a
// RenderShrinkWrappingViewport is given infinite constraints, such as when
// it is the child of a Row or Column (depending on orientation).
//
// For example, a shrink wrapping render sliver may have infinite
// constraints along the viewport's main axis but may also have bouncing
// scroll physics, which will allow for some scrolling effect to occur.
// We should just use the viewportClip - the start of the overlap is at
// double.infinity and so it is effectively meaningless.
if (child.constraints.overlap == 0 || !child.constraints.viewportMainAxisExtent.isFinite) {
return viewportClip;
}
// Adjust the clip rect for this sliver by the overlap from the previous sliver.
double left = viewportClip.left;
double right = viewportClip.right;
double top = viewportClip.top;
double bottom = viewportClip.bottom;
final double startOfOverlap = child.constraints.viewportMainAxisExtent - child.constraints.remainingPaintExtent;
final double overlapCorrection = startOfOverlap + child.constraints.overlap;
switch (applyGrowthDirectionToAxisDirection(axisDirection, child.constraints.growthDirection)) {
case AxisDirection.down:
top += overlapCorrection;
case AxisDirection.up:
bottom -= overlapCorrection;
case AxisDirection.right:
left += overlapCorrection;
case AxisDirection.left:
right -= overlapCorrection;
}
return Rect.fromLTRB(left, top, right, bottom);
}
@override
Rect describeSemanticsClip(RenderSliver? child) {
if (_calculatedCacheExtent == null) {
return semanticBounds;
}
switch (axis) {
case Axis.vertical:
return Rect.fromLTRB(
semanticBounds.left,
semanticBounds.top - _calculatedCacheExtent!,
semanticBounds.right,
semanticBounds.bottom + _calculatedCacheExtent!,
);
case Axis.horizontal:
return Rect.fromLTRB(
semanticBounds.left - _calculatedCacheExtent!,
semanticBounds.top,
semanticBounds.right + _calculatedCacheExtent!,
semanticBounds.bottom,
);
}
}
@override
void paint(PaintingContext context, Offset offset) {
if (firstChild == null) {
return;
}
if (hasVisualOverflow && clipBehavior != Clip.none) {
_clipRectLayer.layer = context.pushClipRect(
needsCompositing,
offset,
Offset.zero & size,
_paintContents,
clipBehavior: clipBehavior,
oldLayer: _clipRectLayer.layer,
);
} else {
_clipRectLayer.layer = null;
_paintContents(context, offset);
}
}
final LayerHandle<ClipRectLayer> _clipRectLayer = LayerHandle<ClipRectLayer>();
@override
void dispose() {
_clipRectLayer.layer = null;
super.dispose();
}
void _paintContents(PaintingContext context, Offset offset) {
for (final RenderSliver child in childrenInPaintOrder) {
if (child.geometry!.visible) {
context.paintChild(child, offset + paintOffsetOf(child));
}
}
}
@override
void debugPaintSize(PaintingContext context, Offset offset) {
assert(() {
super.debugPaintSize(context, offset);
final Paint paint = Paint()
..style = PaintingStyle.stroke
..strokeWidth = 1.0
..color = const Color(0xFF00FF00);
final Canvas canvas = context.canvas;
RenderSliver? child = firstChild;
while (child != null) {
final Size size = switch (axis) {
Axis.vertical => Size(child.constraints.crossAxisExtent, child.geometry!.layoutExtent),
Axis.horizontal => Size(child.geometry!.layoutExtent, child.constraints.crossAxisExtent),
};
canvas.drawRect(((offset + paintOffsetOf(child)) & size).deflate(0.5), paint);
child = childAfter(child);
}
return true;
}());
}
@override
bool hitTestChildren(BoxHitTestResult result, { required Offset position }) {
final (double mainAxisPosition, double crossAxisPosition) = switch (axis) {
Axis.vertical => (position.dy, position.dx),
Axis.horizontal => (position.dx, position.dy),
};
final SliverHitTestResult sliverResult = SliverHitTestResult.wrap(result);
for (final RenderSliver child in childrenInHitTestOrder) {
if (!child.geometry!.visible) {
continue;
}
final Matrix4 transform = Matrix4.identity();
applyPaintTransform(child, transform); // must be invertible
final bool isHit = result.addWithOutOfBandPosition(
paintTransform: transform,
hitTest: (BoxHitTestResult result) {
return child.hitTest(
sliverResult,
mainAxisPosition: computeChildMainAxisPosition(child, mainAxisPosition),
crossAxisPosition: crossAxisPosition,
);
},
);
if (isHit) {
return true;
}
}
return false;
}
@override
RevealedOffset getOffsetToReveal(
RenderObject target,
double alignment, {
Rect? rect,
Axis? axis,
}) {
// One dimensional viewport has only one axis, override if it was
// provided/may be mismatched.
axis = this.axis;
// Steps to convert `rect` (from a RenderBox coordinate system) to its
// scroll offset within this viewport (not in the exact order):
//
// 1. Pick the outermost RenderBox (between which, and the viewport, there
// is nothing but RenderSlivers) as an intermediate reference frame
// (the `pivot`), convert `rect` to that coordinate space.
//
// 2. Convert `rect` from the `pivot` coordinate space to its sliver
// parent's sliver coordinate system (i.e., to a scroll offset), based on
// the axis direction and growth direction of the parent.
//
// 3. Convert the scroll offset to its sliver parent's coordinate space
// using `childScrollOffset`, until we reach the viewport.
//
// 4. Make the final conversion from the outmost sliver to the viewport
// using `scrollOffsetOf`.
double leadingScrollOffset = 0.0;
// Starting at `target` and walking towards the root:
// - `child` will be the last object before we reach this viewport, and
// - `pivot` will be the last RenderBox before we reach this viewport.
RenderObject child = target;
RenderBox? pivot;
bool onlySlivers = target is RenderSliver; // ... between viewport and `target` (`target` included).
while (child.parent != this) {
final RenderObject parent = child.parent!;
if (child is RenderBox) {
pivot = child;
}
if (parent is RenderSliver) {
leadingScrollOffset += parent.childScrollOffset(child)!;
} else {
onlySlivers = false;
leadingScrollOffset = 0.0;
}
child = parent;
}
// `rect` in the new intermediate coordinate system.
final Rect rectLocal;
// Our new reference frame render object's main axis extent.
final double pivotExtent;
final GrowthDirection growthDirection;
// `leadingScrollOffset` is currently the scrollOffset of our new reference
// frame (`pivot` or `target`), within `child`.
if (pivot != null) {
assert(pivot.parent != null);
assert(pivot.parent != this);
assert(pivot != this);
assert(pivot.parent is RenderSliver); // TODO(abarth): Support other kinds of render objects besides slivers.
final RenderSliver pivotParent = pivot.parent! as RenderSliver;
growthDirection = pivotParent.constraints.growthDirection;
pivotExtent = switch (axis) {
Axis.horizontal => pivot.size.width,
Axis.vertical => pivot.size.height,
};
rect ??= target.paintBounds;
rectLocal = MatrixUtils.transformRect(target.getTransformTo(pivot), rect);
} else if (onlySlivers) {
// `pivot` does not exist. We'll have to make up one from `target`, the
// innermost sliver.
final RenderSliver targetSliver = target as RenderSliver;
growthDirection = targetSliver.constraints.growthDirection;
// TODO(LongCatIsLooong): make sure this works if `targetSliver` is a
// persistent header, when #56413 relands.
pivotExtent = targetSliver.geometry!.scrollExtent;
if (rect == null) {
switch (axis) {
case Axis.horizontal:
rect = Rect.fromLTWH(
0, 0,
targetSliver.geometry!.scrollExtent,
targetSliver.constraints.crossAxisExtent,
);
case Axis.vertical:
rect = Rect.fromLTWH(
0, 0,
targetSliver.constraints.crossAxisExtent,
targetSliver.geometry!.scrollExtent,
);
}
}
rectLocal = rect;
} else {
assert(rect != null);
return RevealedOffset(offset: offset.pixels, rect: rect!);
}
assert(child.parent == this);
assert(child is RenderSliver);
final RenderSliver sliver = child as RenderSliver;
// The scroll offset of `rect` within `child`.
leadingScrollOffset += switch (applyGrowthDirectionToAxisDirection(axisDirection, growthDirection)) {
AxisDirection.up => pivotExtent - rectLocal.bottom,
AxisDirection.left => pivotExtent - rectLocal.right,
AxisDirection.right => rectLocal.left,
AxisDirection.down => rectLocal.top,
};
// So far leadingScrollOffset is the scroll offset of `rect` in the `child`
// sliver's sliver coordinate system. The sign of this value indicates
// whether the `rect` protrudes the leading edge of the `child` sliver. When
// this value is non-negative and `child`'s `maxScrollObstructionExtent` is
// greater than 0, we assume `rect` can't be obstructed by the leading edge
// of the viewport (i.e. its pinned to the leading edge).
final bool isPinned = sliver.geometry!.maxScrollObstructionExtent > 0 && leadingScrollOffset >= 0;
// The scroll offset in the viewport to `rect`.
leadingScrollOffset = scrollOffsetOf(sliver, leadingScrollOffset);
// This step assumes the viewport's layout is up-to-date, i.e., if
// offset.pixels is changed after the last performLayout, the new scroll
// position will not be accounted for.
final Matrix4 transform = target.getTransformTo(this);
Rect targetRect = MatrixUtils.transformRect(transform, rect);
final double extentOfPinnedSlivers = maxScrollObstructionExtentBefore(sliver);
switch (sliver.constraints.growthDirection) {
case GrowthDirection.forward:
if (isPinned && alignment <= 0) {
return RevealedOffset(offset: double.infinity, rect: targetRect);
}
leadingScrollOffset -= extentOfPinnedSlivers;
case GrowthDirection.reverse:
if (isPinned && alignment >= 1) {
return RevealedOffset(offset: double.negativeInfinity, rect: targetRect);
}
// If child's growth direction is reverse, when viewport.offset is
// `leadingScrollOffset`, it is positioned just outside of the leading
// edge of the viewport.
leadingScrollOffset -= switch (axis) {
Axis.vertical => targetRect.height,
Axis.horizontal => targetRect.width,
};
}
final double mainAxisExtentDifference = switch (axis) {
Axis.horizontal => size.width - extentOfPinnedSlivers - rectLocal.width,
Axis.vertical => size.height - extentOfPinnedSlivers - rectLocal.height,
};
final double targetOffset = leadingScrollOffset - mainAxisExtentDifference * alignment;
final double offsetDifference = offset.pixels - targetOffset;
targetRect = switch (axisDirection) {
AxisDirection.up => targetRect.translate(0.0, -offsetDifference),
AxisDirection.down => targetRect.translate(0.0, offsetDifference),
AxisDirection.left => targetRect.translate(-offsetDifference, 0.0),
AxisDirection.right => targetRect.translate( offsetDifference, 0.0),
};
return RevealedOffset(offset: targetOffset, rect: targetRect);
}
/// The offset at which the given `child` should be painted.
///
/// The returned offset is from the top left corner of the inside of the
/// viewport to the top left corner of the paint coordinate system of the
/// `child`.
///
/// See also:
///
/// * [paintOffsetOf], which uses the layout offset and growth direction
/// computed for the child during layout.
@protected
Offset computeAbsolutePaintOffset(RenderSliver child, double layoutOffset, GrowthDirection growthDirection) {
assert(hasSize); // this is only usable once we have a size
assert(child.geometry != null);
return switch (applyGrowthDirectionToAxisDirection(axisDirection, growthDirection)) {
AxisDirection.up => Offset(0.0, size.height - layoutOffset - child.geometry!.paintExtent),
AxisDirection.left => Offset(size.width - layoutOffset - child.geometry!.paintExtent, 0.0),
AxisDirection.right => Offset(layoutOffset, 0.0),
AxisDirection.down => Offset(0.0, layoutOffset),
};
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<AxisDirection>('axisDirection', axisDirection));
properties.add(EnumProperty<AxisDirection>('crossAxisDirection', crossAxisDirection));
properties.add(DiagnosticsProperty<ViewportOffset>('offset', offset));
}
@override
List<DiagnosticsNode> debugDescribeChildren() {
final List<DiagnosticsNode> children = <DiagnosticsNode>[];
RenderSliver? child = firstChild;
if (child == null) {
return children;
}
int count = indexOfFirstChild;
while (true) {
children.add(child!.toDiagnosticsNode(name: labelForChild(count)));
if (child == lastChild) {
break;
}
count += 1;
child = childAfter(child);
}
return children;
}
// API TO BE IMPLEMENTED BY SUBCLASSES
// setupParentData
// performLayout (and optionally sizedByParent and performResize)
/// Whether the contents of this viewport would paint outside the bounds of
/// the viewport if [paint] did not clip.
///
/// This property enables an optimization whereby [paint] can skip apply a
/// clip of the contents of the viewport are known to paint entirely within
/// the bounds of the viewport.
@protected
bool get hasVisualOverflow;
/// Called during [layoutChildSequence] for each child.
///
/// Typically used by subclasses to update any out-of-band data, such as the
/// max scroll extent, for each child.
@protected
void updateOutOfBandData(GrowthDirection growthDirection, SliverGeometry childLayoutGeometry);
/// Called during [layoutChildSequence] to store the layout offset for the
/// given child.
///
/// Different subclasses using different representations for their children's
/// layout offset (e.g., logical or physical coordinates). This function lets
/// subclasses transform the child's layout offset before storing it in the
/// child's parent data.
@protected
void updateChildLayoutOffset(RenderSliver child, double layoutOffset, GrowthDirection growthDirection);
/// The offset at which the given `child` should be painted.
///
/// The returned offset is from the top left corner of the inside of the
/// viewport to the top left corner of the paint coordinate system of the
/// `child`.
///
/// See also:
///
/// * [computeAbsolutePaintOffset], which computes the paint offset from an
/// explicit layout offset and growth direction instead of using the values
/// computed for the child during layout.
@protected
Offset paintOffsetOf(RenderSliver child);
/// Returns the scroll offset within the viewport for the given
/// `scrollOffsetWithinChild` within the given `child`.
///
/// The returned value is an estimate that assumes the slivers within the
/// viewport do not change the layout extent in response to changes in their
/// scroll offset.
@protected
double scrollOffsetOf(RenderSliver child, double scrollOffsetWithinChild);
/// Returns the total scroll obstruction extent of all slivers in the viewport
/// before [child].
///
/// This is the extent by which the actual area in which content can scroll
/// is reduced. For example, an app bar that is pinned at the top will reduce
/// the area in which content can actually scroll by the height of the app bar.
@protected
double maxScrollObstructionExtentBefore(RenderSliver child);
/// Converts the `parentMainAxisPosition` into the child's coordinate system.
///
/// The `parentMainAxisPosition` is a distance from the top edge (for vertical
/// viewports) or left edge (for horizontal viewports) of the viewport bounds.
/// This describes a line, perpendicular to the viewport's main axis, heretofore
/// known as the target line.
///
/// The child's coordinate system's origin in the main axis is at the leading
/// edge of the given child, as given by the child's
/// [SliverConstraints.axisDirection] and [SliverConstraints.growthDirection].
///
/// This method returns the distance from the leading edge of the given child to
/// the target line described above.
///
/// (The `parentMainAxisPosition` is not from the leading edge of the
/// viewport, it's always the top or left edge.)
@protected
double computeChildMainAxisPosition(RenderSliver child, double parentMainAxisPosition);
/// The index of the first child of the viewport relative to the center child.
///
/// For example, the center child has index zero and the first child in the
/// reverse growth direction has index -1.
@protected
int get indexOfFirstChild;
/// A short string to identify the child with the given index.
///
/// Used by [debugDescribeChildren] to label the children.
@protected
String labelForChild(int index);
/// Provides an iterable that walks the children of the viewport, in the order
/// that they should be painted.
///
/// This should be the reverse order of [childrenInHitTestOrder].
@protected
Iterable<RenderSliver> get childrenInPaintOrder;
/// Provides an iterable that walks the children of the viewport, in the order
/// that hit-testing should use.
///
/// This should be the reverse order of [childrenInPaintOrder].
@protected
Iterable<RenderSliver> get childrenInHitTestOrder;
@override
void showOnScreen({
RenderObject? descendant,
Rect? rect,
Duration duration = Duration.zero,
Curve curve = Curves.ease,
}) {
if (!offset.allowImplicitScrolling) {
return super.showOnScreen(
descendant: descendant,
rect: rect,
duration: duration,
curve: curve,
);
}
final Rect? newRect = RenderViewportBase.showInViewport(
descendant: descendant,
viewport: this,
offset: offset,
rect: rect,
duration: duration,
curve: curve,
);
super.showOnScreen(
rect: newRect,
duration: duration,
curve: curve,
);
}
/// Make (a portion of) the given `descendant` of the given `viewport` fully
/// visible in the `viewport` by manipulating the provided [ViewportOffset]
/// `offset`.
///
/// The optional `rect` parameter describes which area of the `descendant`
/// should be shown in the viewport. If `rect` is null, the entire
/// `descendant` will be revealed. The `rect` parameter is interpreted
/// relative to the coordinate system of `descendant`.
///
/// The returned [Rect] describes the new location of `descendant` or `rect`
/// in the viewport after it has been revealed. See [RevealedOffset.rect]
/// for a full definition of this [Rect].
///
/// If `descendant` is null, this is a no-op and `rect` is returned.
///
/// If both `descendant` and `rect` are null, null is returned because there is
/// nothing to be shown in the viewport.
///
/// The `duration` parameter can be set to a non-zero value to animate the
/// target object into the viewport with an animation defined by `curve`.
///
/// See also:
///
/// * [RenderObject.showOnScreen], overridden by [RenderViewportBase] and the
/// renderer for [SingleChildScrollView] to delegate to this method.
static Rect? showInViewport({
RenderObject? descendant,
Rect? rect,
required RenderAbstractViewport viewport,
required ViewportOffset offset,
Duration duration = Duration.zero,
Curve curve = Curves.ease,
}) {
if (descendant == null) {
return rect;
}
final RevealedOffset leadingEdgeOffset = viewport.getOffsetToReveal(descendant, 0.0, rect: rect);
final RevealedOffset trailingEdgeOffset = viewport.getOffsetToReveal(descendant, 1.0, rect: rect);
final double currentOffset = offset.pixels;
final RevealedOffset? targetOffset = RevealedOffset.clampOffset(
leadingEdgeOffset: leadingEdgeOffset,
trailingEdgeOffset: trailingEdgeOffset,
currentOffset: currentOffset,
);
if (targetOffset == null) {
// `descendant` is between leading and trailing edge and hence already
// fully shown on screen. No action necessary.
assert(viewport.parent != null);
final Matrix4 transform = descendant.getTransformTo(viewport.parent);
return MatrixUtils.transformRect(transform, rect ?? descendant.paintBounds);
}
offset.moveTo(targetOffset.offset, duration: duration, curve: curve);
return targetOffset.rect;
}
}
/// A render object that is bigger on the inside.
///
/// [RenderViewport] is the visual workhorse of the scrolling machinery. It
/// displays a subset of its children according to its own dimensions and the
/// given [offset]. As the offset varies, different children are visible through
/// the viewport.
///
/// [RenderViewport] hosts a bidirectional list of slivers in a single shared
/// [Axis], anchored on a [center] sliver, which is placed at the zero scroll
/// offset. The center widget is displayed in the viewport according to the
/// [anchor] property.
///
/// Slivers that are earlier in the child list than [center] are displayed in
/// reverse order in the reverse [axisDirection] starting from the [center]. For
/// example, if the [axisDirection] is [AxisDirection.down], the first sliver
/// before [center] is placed above the [center]. The slivers that are later in
/// the child list than [center] are placed in order in the [axisDirection]. For
/// example, in the preceding scenario, the first sliver after [center] is
/// placed below the [center].
///
/// {@macro flutter.rendering.GrowthDirection.sample}
///
/// [RenderViewport] cannot contain [RenderBox] children directly. Instead, use
/// a [RenderSliverList], [RenderSliverFixedExtentList], [RenderSliverGrid], or
/// a [RenderSliverToBoxAdapter], for example.
///
/// See also:
///
/// * [RenderSliver], which explains more about the Sliver protocol.
/// * [RenderBox], which explains more about the Box protocol.
/// * [RenderSliverToBoxAdapter], which allows a [RenderBox] object to be
/// placed inside a [RenderSliver] (the opposite of this class).
/// * [RenderShrinkWrappingViewport], a variant of [RenderViewport] that
/// shrink-wraps its contents along the main axis.
class RenderViewport extends RenderViewportBase<SliverPhysicalContainerParentData> {
/// Creates a viewport for [RenderSliver] objects.
///
/// If the [center] is not specified, then the first child in the `children`
/// list, if any, is used.
///
/// The [offset] must be specified. For testing purposes, consider passing a
/// [ViewportOffset.zero] or [ViewportOffset.fixed].
RenderViewport({
super.axisDirection,
required super.crossAxisDirection,
required super.offset,
double anchor = 0.0,
List<RenderSliver>? children,
RenderSliver? center,
super.cacheExtent,
super.cacheExtentStyle,
super.clipBehavior,
}) : assert(anchor >= 0.0 && anchor <= 1.0),
assert(cacheExtentStyle != CacheExtentStyle.viewport || cacheExtent != null),
_anchor = anchor,
_center = center {
addAll(children);
if (center == null && firstChild != null) {
_center = firstChild;
}
}
/// If a [RenderAbstractViewport] overrides
/// [RenderObject.describeSemanticsConfiguration] to add the [SemanticsTag]
/// [useTwoPaneSemantics] to its [SemanticsConfiguration], two semantics nodes
/// will be used to represent the viewport with its associated scrolling
/// actions in the semantics tree.
///
/// Two semantics nodes (an inner and an outer node) are necessary to exclude
/// certain child nodes (via the [excludeFromScrolling] tag) from the
/// scrollable area for semantic purposes: The [SemanticsNode]s of children
/// that should be excluded from scrolling will be attached to the outer node.
/// The semantic scrolling actions and the [SemanticsNode]s of scrollable
/// children will be attached to the inner node, which itself is a child of
/// the outer node.
///
/// See also:
///
/// * [RenderViewportBase.describeSemanticsConfiguration], which adds this
/// tag to its [SemanticsConfiguration].
static const SemanticsTag useTwoPaneSemantics = SemanticsTag('RenderViewport.twoPane');
/// When a top-level [SemanticsNode] below a [RenderAbstractViewport] is
/// tagged with [excludeFromScrolling] it will not be part of the scrolling
/// area for semantic purposes.
///
/// This behavior is only active if the [RenderAbstractViewport]
/// tagged its [SemanticsConfiguration] with [useTwoPaneSemantics].
/// Otherwise, the [excludeFromScrolling] tag is ignored.
///
/// As an example, a [RenderSliver] that stays on the screen within a
/// [Scrollable] even though the user has scrolled past it (e.g. a pinned app
/// bar) can tag its [SemanticsNode] with [excludeFromScrolling] to indicate
/// that it should no longer be considered for semantic actions related to
/// scrolling.
static const SemanticsTag excludeFromScrolling = SemanticsTag('RenderViewport.excludeFromScrolling');
@override
void setupParentData(RenderObject child) {
if (child.parentData is! SliverPhysicalContainerParentData) {
child.parentData = SliverPhysicalContainerParentData();
}
}
/// The relative position of the zero scroll offset.
///
/// For example, if [anchor] is 0.5 and the [axisDirection] is
/// [AxisDirection.down] or [AxisDirection.up], then the zero scroll offset is
/// vertically centered within the viewport. If the [anchor] is 1.0, and the
/// [axisDirection] is [AxisDirection.right], then the zero scroll offset is
/// on the left edge of the viewport.
///
/// {@macro flutter.rendering.GrowthDirection.sample}
double get anchor => _anchor;
double _anchor;
set anchor(double value) {
assert(value >= 0.0 && value <= 1.0);
if (value == _anchor) {
return;
}
_anchor = value;
markNeedsLayout();
}
/// The first child in the [GrowthDirection.forward] growth direction.
///
/// This child that will be at the position defined by [anchor] when the
/// [ViewportOffset.pixels] of [offset] is `0`.
///
/// Children after [center] will be placed in the [axisDirection] relative to
/// the [center].
///
/// Children before [center] will be placed in the opposite of
/// the [axisDirection] relative to the [center]. These children above
/// [center] will have a growth direction of [GrowthDirection.reverse].
///
/// The [center] must be a direct child of the viewport.
///
/// {@macro flutter.rendering.GrowthDirection.sample}
RenderSliver? get center => _center;
RenderSliver? _center;
set center(RenderSliver? value) {
if (value == _center) {
return;
}
_center = value;
markNeedsLayout();
}
@override
bool get sizedByParent => true;
@override
@protected
Size computeDryLayout(covariant BoxConstraints constraints) {
assert(debugCheckHasBoundedAxis(axis, constraints));
return constraints.biggest;
}
static const int _maxLayoutCyclesPerChild = 10;
// Out-of-band data computed during layout.
late double _minScrollExtent;
late double _maxScrollExtent;
bool _hasVisualOverflow = false;
@override
void performLayout() {
// Ignore the return value of applyViewportDimension because we are
// doing a layout regardless.
switch (axis) {
case Axis.vertical:
offset.applyViewportDimension(size.height);
case Axis.horizontal:
offset.applyViewportDimension(size.width);
}
if (center == null) {
assert(firstChild == null);
_minScrollExtent = 0.0;
_maxScrollExtent = 0.0;
_hasVisualOverflow = false;
offset.applyContentDimensions(0.0, 0.0);
return;
}
assert(center!.parent == this);
final (double mainAxisExtent, double crossAxisExtent) = switch (axis) {
Axis.vertical => (size.height, size.width),
Axis.horizontal => (size.width, size.height),
};
final double centerOffsetAdjustment = center!.centerOffsetAdjustment;
final int maxLayoutCycles = _maxLayoutCyclesPerChild * childCount;
double correction;
int count = 0;
do {
correction = _attemptLayout(mainAxisExtent, crossAxisExtent, offset.pixels + centerOffsetAdjustment);
if (correction != 0.0) {
offset.correctBy(correction);
} else {
if (offset.applyContentDimensions(
math.min(0.0, _minScrollExtent + mainAxisExtent * anchor),
math.max(0.0, _maxScrollExtent - mainAxisExtent * (1.0 - anchor)),
)) {
break;
}
}
count += 1;
} while (count < maxLayoutCycles);
assert(() {
if (count >= maxLayoutCycles) {
assert(count != 1);
throw FlutterError(
'A RenderViewport exceeded its maximum number of layout cycles.\n'
'RenderViewport render objects, during layout, can retry if either their '
'slivers or their ViewportOffset decide that the offset should be corrected '
'to take into account information collected during that layout.\n'
'In the case of this RenderViewport object, however, this happened $count '
'times and still there was no consensus on the scroll offset. This usually '
'indicates a bug. Specifically, it means that one of the following three '
'problems is being experienced by the RenderViewport object:\n'
' * One of the RenderSliver children or the ViewportOffset have a bug such'
' that they always think that they need to correct the offset regardless.\n'
' * Some combination of the RenderSliver children and the ViewportOffset'
' have a bad interaction such that one applies a correction then another'
' applies a reverse correction, leading to an infinite loop of corrections.\n'
' * There is a pathological case that would eventually resolve, but it is'
' so complicated that it cannot be resolved in any reasonable number of'
' layout passes.',
);
}
return true;
}());
}
double _attemptLayout(double mainAxisExtent, double crossAxisExtent, double correctedOffset) {
assert(!mainAxisExtent.isNaN);
assert(mainAxisExtent >= 0.0);
assert(crossAxisExtent.isFinite);
assert(crossAxisExtent >= 0.0);
assert(correctedOffset.isFinite);
_minScrollExtent = 0.0;
_maxScrollExtent = 0.0;
_hasVisualOverflow = false;
// centerOffset is the offset from the leading edge of the RenderViewport
// to the zero scroll offset (the line between the forward slivers and the
// reverse slivers).
final double centerOffset = mainAxisExtent * anchor - correctedOffset;
final double reverseDirectionRemainingPaintExtent = clampDouble(centerOffset, 0.0, mainAxisExtent);
final double forwardDirectionRemainingPaintExtent = clampDouble(mainAxisExtent - centerOffset, 0.0, mainAxisExtent);
_calculatedCacheExtent = switch (cacheExtentStyle) {
CacheExtentStyle.pixel => cacheExtent,
CacheExtentStyle.viewport => mainAxisExtent * _cacheExtent,
};
final double fullCacheExtent = mainAxisExtent + 2 * _calculatedCacheExtent!;
final double centerCacheOffset = centerOffset + _calculatedCacheExtent!;
final double reverseDirectionRemainingCacheExtent = clampDouble(centerCacheOffset, 0.0, fullCacheExtent);
final double forwardDirectionRemainingCacheExtent = clampDouble(fullCacheExtent - centerCacheOffset, 0.0, fullCacheExtent);
final RenderSliver? leadingNegativeChild = childBefore(center!);
if (leadingNegativeChild != null) {
// negative scroll offsets
final double result = layoutChildSequence(
child: leadingNegativeChild,
scrollOffset: math.max(mainAxisExtent, centerOffset) - mainAxisExtent,
overlap: 0.0,
layoutOffset: forwardDirectionRemainingPaintExtent,
remainingPaintExtent: reverseDirectionRemainingPaintExtent,
mainAxisExtent: mainAxisExtent,
crossAxisExtent: crossAxisExtent,
growthDirection: GrowthDirection.reverse,
advance: childBefore,
remainingCacheExtent: reverseDirectionRemainingCacheExtent,
cacheOrigin: clampDouble(mainAxisExtent - centerOffset, -_calculatedCacheExtent!, 0.0),
);
if (result != 0.0) {
return -result;
}
}
// positive scroll offsets
return layoutChildSequence(
child: center,
scrollOffset: math.max(0.0, -centerOffset),
overlap: leadingNegativeChild == null ? math.min(0.0, -centerOffset) : 0.0,
layoutOffset: centerOffset >= mainAxisExtent ? centerOffset: reverseDirectionRemainingPaintExtent,
remainingPaintExtent: forwardDirectionRemainingPaintExtent,
mainAxisExtent: mainAxisExtent,
crossAxisExtent: crossAxisExtent,
growthDirection: GrowthDirection.forward,
advance: childAfter,
remainingCacheExtent: forwardDirectionRemainingCacheExtent,
cacheOrigin: clampDouble(centerOffset, -_calculatedCacheExtent!, 0.0),
);
}
@override
bool get hasVisualOverflow => _hasVisualOverflow;
@override
void updateOutOfBandData(GrowthDirection growthDirection, SliverGeometry childLayoutGeometry) {
switch (growthDirection) {
case GrowthDirection.forward:
_maxScrollExtent += childLayoutGeometry.scrollExtent;
case GrowthDirection.reverse:
_minScrollExtent -= childLayoutGeometry.scrollExtent;
}
if (childLayoutGeometry.hasVisualOverflow) {
_hasVisualOverflow = true;
}
}
@override
void updateChildLayoutOffset(RenderSliver child, double layoutOffset, GrowthDirection growthDirection) {
final SliverPhysicalParentData childParentData = child.parentData! as SliverPhysicalParentData;
childParentData.paintOffset = computeAbsolutePaintOffset(child, layoutOffset, growthDirection);
}
@override
Offset paintOffsetOf(RenderSliver child) {
final SliverPhysicalParentData childParentData = child.parentData! as SliverPhysicalParentData;
return childParentData.paintOffset;
}
@override
double scrollOffsetOf(RenderSliver child, double scrollOffsetWithinChild) {
assert(child.parent == this);
final GrowthDirection growthDirection = child.constraints.growthDirection;
switch (growthDirection) {
case GrowthDirection.forward:
double scrollOffsetToChild = 0.0;
RenderSliver? current = center;
while (current != child) {
scrollOffsetToChild += current!.geometry!.scrollExtent;
current = childAfter(current);
}
return scrollOffsetToChild + scrollOffsetWithinChild;
case GrowthDirection.reverse:
double scrollOffsetToChild = 0.0;
RenderSliver? current = childBefore(center!);
while (current != child) {
scrollOffsetToChild -= current!.geometry!.scrollExtent;
current = childBefore(current);
}
return scrollOffsetToChild - scrollOffsetWithinChild;
}
}
@override
double maxScrollObstructionExtentBefore(RenderSliver child) {
assert(child.parent == this);
final GrowthDirection growthDirection = child.constraints.growthDirection;
switch (growthDirection) {
case GrowthDirection.forward:
double pinnedExtent = 0.0;
RenderSliver? current = center;
while (current != child) {
pinnedExtent += current!.geometry!.maxScrollObstructionExtent;
current = childAfter(current);
}
return pinnedExtent;
case GrowthDirection.reverse:
double pinnedExtent = 0.0;
RenderSliver? current = childBefore(center!);
while (current != child) {
pinnedExtent += current!.geometry!.maxScrollObstructionExtent;
current = childBefore(current);
}
return pinnedExtent;
}
}
@override
void applyPaintTransform(RenderObject child, Matrix4 transform) {
// Hit test logic relies on this always providing an invertible matrix.
final SliverPhysicalParentData childParentData = child.parentData! as SliverPhysicalParentData;
childParentData.applyPaintTransform(transform);
}
@override
double computeChildMainAxisPosition(RenderSliver child, double parentMainAxisPosition) {
final Offset paintOffset = (child.parentData! as SliverPhysicalParentData).paintOffset;
return switch (applyGrowthDirectionToAxisDirection(child.constraints.axisDirection, child.constraints.growthDirection)) {
AxisDirection.down => parentMainAxisPosition - paintOffset.dy,
AxisDirection.right => parentMainAxisPosition - paintOffset.dx,
AxisDirection.up => child.geometry!.paintExtent - (parentMainAxisPosition - paintOffset.dy),
AxisDirection.left => child.geometry!.paintExtent - (parentMainAxisPosition - paintOffset.dx),
};
}
@override
int get indexOfFirstChild {
assert(center != null);
assert(center!.parent == this);
assert(firstChild != null);
int count = 0;
RenderSliver? child = center;
while (child != firstChild) {
count -= 1;
child = childBefore(child!);
}
return count;
}
@override
String labelForChild(int index) {
if (index == 0) {
return 'center child';
}
return 'child $index';
}
@override
Iterable<RenderSliver> get childrenInPaintOrder {
final List<RenderSliver> children = <RenderSliver>[];
if (firstChild == null) {
return children;
}
RenderSliver? child = firstChild;
while (child != center) {
children.add(child!);
child = childAfter(child);
}
child = lastChild;
while (true) {
children.add(child!);
if (child == center) {
return children;
}
child = childBefore(child);
}
}
@override
Iterable<RenderSliver> get childrenInHitTestOrder {
final List<RenderSliver> children = <RenderSliver>[];
if (firstChild == null) {
return children;
}
RenderSliver? child = center;
while (child != null) {
children.add(child);
child = childAfter(child);
}
child = childBefore(center!);
while (child != null) {
children.add(child);
child = childBefore(child);
}
return children;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('anchor', anchor));
}
}
/// A render object that is bigger on the inside and shrink wraps its children
/// in the main axis.
///
/// [RenderShrinkWrappingViewport] displays a subset of its children according
/// to its own dimensions and the given [offset]. As the offset varies, different
/// children are visible through the viewport.
///
/// [RenderShrinkWrappingViewport] differs from [RenderViewport] in that
/// [RenderViewport] expands to fill the main axis whereas
/// [RenderShrinkWrappingViewport] sizes itself to match its children in the
/// main axis. This shrink wrapping behavior is expensive because the children,
/// and hence the viewport, could potentially change size whenever the [offset]
/// changes (e.g., because of a collapsing header).
///
/// [RenderShrinkWrappingViewport] cannot contain [RenderBox] children directly.
/// Instead, use a [RenderSliverList], [RenderSliverFixedExtentList],
/// [RenderSliverGrid], or a [RenderSliverToBoxAdapter], for example.
///
/// See also:
///
/// * [RenderViewport], a viewport that does not shrink-wrap its contents.
/// * [RenderSliver], which explains more about the Sliver protocol.
/// * [RenderBox], which explains more about the Box protocol.
/// * [RenderSliverToBoxAdapter], which allows a [RenderBox] object to be
/// placed inside a [RenderSliver] (the opposite of this class).
class RenderShrinkWrappingViewport extends RenderViewportBase<SliverLogicalContainerParentData> {
/// Creates a viewport (for [RenderSliver] objects) that shrink-wraps its
/// contents.
///
/// The [offset] must be specified. For testing purposes, consider passing a
/// [ViewportOffset.zero] or [ViewportOffset.fixed].
RenderShrinkWrappingViewport({
super.axisDirection,
required super.crossAxisDirection,
required super.offset,
super.clipBehavior,
List<RenderSliver>? children,
}) {
addAll(children);
}
@override
void setupParentData(RenderObject child) {
if (child.parentData is! SliverLogicalContainerParentData) {
child.parentData = SliverLogicalContainerParentData();
}
}
@override
bool debugThrowIfNotCheckingIntrinsics() {
assert(() {
if (!RenderObject.debugCheckingIntrinsics) {
throw FlutterError.fromParts(<DiagnosticsNode>[
ErrorSummary('$runtimeType does not support returning intrinsic dimensions.'),
ErrorDescription(
'Calculating the intrinsic dimensions would require instantiating every child of '
'the viewport, which defeats the point of viewports being lazy.',
),
ErrorHint(
'If you are merely trying to shrink-wrap the viewport in the main axis direction, '
'you should be able to achieve that effect by just giving the viewport loose '
'constraints, without needing to measure its intrinsic dimensions.',
),
]);
}
return true;
}());
return true;
}
// Out-of-band data computed during layout.
late double _maxScrollExtent;
late double _shrinkWrapExtent;
bool _hasVisualOverflow = false;
bool _debugCheckHasBoundedCrossAxis() {
assert(() {
switch (axis) {
case Axis.vertical:
if (!constraints.hasBoundedWidth) {
throw FlutterError(
'Vertical viewport was given unbounded width.\n'
'Viewports expand in the cross axis to fill their container and '
'constrain their children to match their extent in the cross axis. '
'In this case, a vertical shrinkwrapping viewport was given an '
'unlimited amount of horizontal space in which to expand.',
);
}
case Axis.horizontal:
if (!constraints.hasBoundedHeight) {
throw FlutterError(
'Horizontal viewport was given unbounded height.\n'
'Viewports expand in the cross axis to fill their container and '
'constrain their children to match their extent in the cross axis. '
'In this case, a horizontal shrinkwrapping viewport was given an '
'unlimited amount of vertical space in which to expand.',
);
}
}
return true;
}());
return true;
}
@override
void performLayout() {
final BoxConstraints constraints = this.constraints;
if (firstChild == null) {
// Shrinkwrapping viewport only requires the cross axis to be bounded.
assert(_debugCheckHasBoundedCrossAxis());
size = switch (axis) {
Axis.vertical => Size(constraints.maxWidth, constraints.minHeight),
Axis.horizontal => Size(constraints.minWidth, constraints.maxHeight),
};
offset.applyViewportDimension(0.0);
_maxScrollExtent = 0.0;
_shrinkWrapExtent = 0.0;
_hasVisualOverflow = false;
offset.applyContentDimensions(0.0, 0.0);
return;
}
// Shrinkwrapping viewport only requires the cross axis to be bounded.
assert(_debugCheckHasBoundedCrossAxis());
final (double mainAxisExtent, double crossAxisExtent) = switch (axis) {
Axis.vertical => (constraints.maxHeight, constraints.maxWidth),
Axis.horizontal => (constraints.maxWidth, constraints.maxHeight),
};
double correction;
double effectiveExtent;
while (true) {
correction = _attemptLayout(mainAxisExtent, crossAxisExtent, offset.pixels);
if (correction != 0.0) {
offset.correctBy(correction);
} else {
effectiveExtent = switch (axis) {
Axis.vertical => constraints.constrainHeight(_shrinkWrapExtent),
Axis.horizontal => constraints.constrainWidth(_shrinkWrapExtent),
};
final bool didAcceptViewportDimension = offset.applyViewportDimension(effectiveExtent);
final bool didAcceptContentDimension = offset.applyContentDimensions(0.0, math.max(0.0, _maxScrollExtent - effectiveExtent));
if (didAcceptViewportDimension && didAcceptContentDimension) {
break;
}
}
}
size = switch (axis) {
Axis.vertical => constraints.constrainDimensions(crossAxisExtent, effectiveExtent),
Axis.horizontal => constraints.constrainDimensions(effectiveExtent, crossAxisExtent),
};
}
double _attemptLayout(double mainAxisExtent, double crossAxisExtent, double correctedOffset) {
// We can't assert mainAxisExtent is finite, because it could be infinite if
// it is within a column or row for example. In such a case, there's not
// even any scrolling to do, although some scroll physics (i.e.
// BouncingScrollPhysics) could still temporarily scroll the content in a
// simulation.
assert(!mainAxisExtent.isNaN);
assert(mainAxisExtent >= 0.0);
assert(crossAxisExtent.isFinite);
assert(crossAxisExtent >= 0.0);
assert(correctedOffset.isFinite);
_maxScrollExtent = 0.0;
_shrinkWrapExtent = 0.0;
// Since the viewport is shrinkwrapped, we know that any negative overscroll
// into the potentially infinite mainAxisExtent will overflow the end of
// the viewport.
_hasVisualOverflow = correctedOffset < 0.0;
_calculatedCacheExtent = switch (cacheExtentStyle) {
CacheExtentStyle.pixel => cacheExtent,
CacheExtentStyle.viewport => mainAxisExtent * _cacheExtent,
};
return layoutChildSequence(
child: firstChild,
scrollOffset: math.max(0.0, correctedOffset),
overlap: math.min(0.0, correctedOffset),
layoutOffset: math.max(0.0, -correctedOffset),
remainingPaintExtent: mainAxisExtent + math.min(0.0, correctedOffset),
mainAxisExtent: mainAxisExtent,
crossAxisExtent: crossAxisExtent,
growthDirection: GrowthDirection.forward,
advance: childAfter,
remainingCacheExtent: mainAxisExtent + 2 * _calculatedCacheExtent!,
cacheOrigin: -_calculatedCacheExtent!,
);
}
@override
bool get hasVisualOverflow => _hasVisualOverflow;
@override
void updateOutOfBandData(GrowthDirection growthDirection, SliverGeometry childLayoutGeometry) {
assert(growthDirection == GrowthDirection.forward);
_maxScrollExtent += childLayoutGeometry.scrollExtent;
if (childLayoutGeometry.hasVisualOverflow) {
_hasVisualOverflow = true;
}
_shrinkWrapExtent += childLayoutGeometry.maxPaintExtent;
}
@override
void updateChildLayoutOffset(RenderSliver child, double layoutOffset, GrowthDirection growthDirection) {
assert(growthDirection == GrowthDirection.forward);
final SliverLogicalParentData childParentData = child.parentData! as SliverLogicalParentData;
childParentData.layoutOffset = layoutOffset;
}
@override
Offset paintOffsetOf(RenderSliver child) {
final SliverLogicalParentData childParentData = child.parentData! as SliverLogicalParentData;
return computeAbsolutePaintOffset(child, childParentData.layoutOffset!, GrowthDirection.forward);
}
@override
double scrollOffsetOf(RenderSliver child, double scrollOffsetWithinChild) {
assert(child.parent == this);
assert(child.constraints.growthDirection == GrowthDirection.forward);
double scrollOffsetToChild = 0.0;
RenderSliver? current = firstChild;
while (current != child) {
scrollOffsetToChild += current!.geometry!.scrollExtent;
current = childAfter(current);
}
return scrollOffsetToChild + scrollOffsetWithinChild;
}
@override
double maxScrollObstructionExtentBefore(RenderSliver child) {
assert(child.parent == this);
assert(child.constraints.growthDirection == GrowthDirection.forward);
double pinnedExtent = 0.0;
RenderSliver? current = firstChild;
while (current != child) {
pinnedExtent += current!.geometry!.maxScrollObstructionExtent;
current = childAfter(current);
}
return pinnedExtent;
}
@override
void applyPaintTransform(RenderObject child, Matrix4 transform) {
// Hit test logic relies on this always providing an invertible matrix.
final Offset offset = paintOffsetOf(child as RenderSliver);
transform.translate(offset.dx, offset.dy);
}
@override
double computeChildMainAxisPosition(RenderSliver child, double parentMainAxisPosition) {
assert(hasSize);
final double layoutOffset = (child.parentData! as SliverLogicalParentData).layoutOffset!;
return switch (applyGrowthDirectionToAxisDirection(child.constraints.axisDirection, child.constraints.growthDirection)) {
AxisDirection.down || AxisDirection.right => parentMainAxisPosition - layoutOffset,
AxisDirection.up => size.height - parentMainAxisPosition - layoutOffset,
AxisDirection.left => size.width - parentMainAxisPosition - layoutOffset,
};
}
@override
int get indexOfFirstChild => 0;
@override
String labelForChild(int index) => 'child $index';
@override
Iterable<RenderSliver> get childrenInPaintOrder {
final List<RenderSliver> children = <RenderSliver>[];
RenderSliver? child = lastChild;
while (child != null) {
children.add(child);
child = childBefore(child);
}
return children;
}
@override
Iterable<RenderSliver> get childrenInHitTestOrder {
final List<RenderSliver> children = <RenderSliver>[];
RenderSliver? child = firstChild;
while (child != null) {
children.add(child);
child = childAfter(child);
}
return children;
}
}
| flutter/packages/flutter/lib/src/rendering/viewport.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/rendering/viewport.dart",
"repo_id": "flutter",
"token_count": 25742
} | 665 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:convert';
import 'package:flutter/foundation.dart';
import 'asset_bundle.dart';
import 'message_codecs.dart';
// We use .bin as the extension since it is well-known to represent
// data in some arbitrary binary format.
const String _kAssetManifestFilename = 'AssetManifest.bin';
// We use the same bin file for the web, but re-encoded as JSON(base64(bytes))
// so it can be downloaded by even the dumbest of browsers.
// See https://github.com/flutter/flutter/issues/128456
const String _kAssetManifestWebFilename = 'AssetManifest.bin.json';
/// Contains details about available assets and their variants.
/// See [Resolution-aware image assets](https://docs.flutter.dev/ui/assets-and-images#resolution-aware)
/// to learn about asset variants and how to declare them.
abstract class AssetManifest {
/// Loads asset manifest data from an [AssetBundle] object and creates an
/// [AssetManifest] object from that data.
static Future<AssetManifest> loadFromAssetBundle(AssetBundle bundle) {
// The AssetManifest file contains binary data.
//
// On the web, the build process wraps this binary data in json+base64 so
// it can be transmitted over the network without special configuration
// (see #131382).
if (kIsWeb) {
// On the web, the AssetManifest is downloaded as a String, then
// json+base64-decoded to get to the binary data.
return bundle.loadStructuredData(_kAssetManifestWebFilename, (String jsonData) async {
// Decode the manifest JSON file to the underlying BIN, and convert to ByteData.
final ByteData message = ByteData.sublistView(base64.decode(json.decode(jsonData) as String));
// Now we can keep operating as usual.
return _AssetManifestBin.fromStandardMessageCodecMessage(message);
});
}
// On every other platform, the binary file contents are used directly.
return bundle.loadStructuredBinaryData(_kAssetManifestFilename, _AssetManifestBin.fromStandardMessageCodecMessage);
}
/// Lists the keys of all main assets. This does not include assets
/// that are variants of other assets.
///
/// The logical key maps to the path of an asset specified in the pubspec.yaml
/// file at build time.
///
/// See [Specifying assets](https://docs.flutter.dev/development/ui/assets-and-images#specifying-assets)
/// and [Loading assets](https://docs.flutter.dev/development/ui/assets-and-images#loading-assets)
/// for more information.
List<String> listAssets();
/// Retrieves metadata about an asset and its variants. Returns null if the
/// key was not found in the asset manifest.
///
/// This method considers a main asset to be a variant of itself. The returned
/// list will include it if it exists.
List<AssetMetadata>? getAssetVariants(String key);
}
// Lazily parses the binary asset manifest into a data structure that's easier to work
// with.
//
// The binary asset manifest is a map of asset keys to a list of objects
// representing the asset's variants.
//
// The entries with each variant object are:
// - "asset": the location of this variant to load it from.
// - "dpr": The device-pixel-ratio that the asset is best-suited for.
//
// New fields could be added to this object schema to support new asset variation
// features, such as themes, locale/region support, reading directions, and so on.
class _AssetManifestBin implements AssetManifest {
_AssetManifestBin(Map<Object?, Object?> standardMessageData): _data = standardMessageData;
factory _AssetManifestBin.fromStandardMessageCodecMessage(ByteData message) {
final dynamic data = const StandardMessageCodec().decodeMessage(message);
return _AssetManifestBin(data as Map<Object?, Object?>);
}
final Map<Object?, Object?> _data;
final Map<String, List<AssetMetadata>> _typeCastedData = <String, List<AssetMetadata>>{};
@override
List<AssetMetadata>? getAssetVariants(String key) {
// We lazily delay typecasting to prevent a performance hiccup when parsing
// large asset manifests. This is important to keep an app's first asset
// load fast.
if (!_typeCastedData.containsKey(key)) {
final Object? variantData = _data[key];
if (variantData == null) {
return null;
}
_typeCastedData[key] = ((_data[key] ?? <Object?>[]) as Iterable<Object?>)
.cast<Map<Object?, Object?>>()
.map((Map<Object?, Object?> data) {
final String asset = data['asset']! as String;
final Object? dpr = data['dpr'];
return AssetMetadata(
key: data['asset']! as String,
targetDevicePixelRatio: dpr as double?,
main: key == asset,
);
})
.toList();
_data.remove(key);
}
return _typeCastedData[key]!;
}
@override
List<String> listAssets() {
return <String>[..._data.keys.cast<String>(), ..._typeCastedData.keys];
}
}
/// Contains information about an asset.
@immutable
class AssetMetadata {
/// Creates an object containing information about an asset.
const AssetMetadata({
required this.key,
required this.targetDevicePixelRatio,
required this.main,
});
/// The device pixel ratio that this asset is most ideal for. This is determined
/// by the name of the parent folder of the asset file. For example, if the
/// parent folder is named "3.0x", the target device pixel ratio of that
/// asset will be interpreted as 3.
///
/// This will be null if the parent folder name is not a ratio value followed
/// by an "x".
///
/// See [Resolution-aware image assets](https://docs.flutter.dev/development/ui/assets-and-images#resolution-aware)
/// for more information.
final double? targetDevicePixelRatio;
/// The asset's key, which is the path to the asset specified in the pubspec.yaml
/// file at build time.
final String key;
/// Whether or not this is a main asset. In other words, this is true if
/// this asset is not a variant of another asset.
final bool main;
}
| flutter/packages/flutter/lib/src/services/asset_manifest.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/asset_manifest.dart",
"repo_id": "flutter",
"token_count": 1930
} | 666 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'platform_channel.dart';
export 'dart:typed_data' show ByteData;
/// A message encoding/decoding mechanism.
///
/// Both operations throw an exception, if conversion fails. Such situations
/// should be treated as programming errors.
///
/// See also:
///
/// * [BasicMessageChannel], which use [MessageCodec]s for communication
/// between Flutter and platform plugins.
abstract class MessageCodec<T> {
/// Encodes the specified [message] in binary.
///
/// Returns null if the message is null.
ByteData? encodeMessage(T message);
/// Decodes the specified [message] from binary.
///
/// Returns null if the message is null.
T? decodeMessage(ByteData? message);
}
/// A command object representing the invocation of a named method.
@pragma('vm:keep-name')
@immutable
class MethodCall {
/// Creates a [MethodCall] representing the invocation of [method] with the
/// specified [arguments].
const MethodCall(this.method, [this.arguments]);
/// The name of the method to be called.
final String method;
/// The arguments for the method.
///
/// Must be a valid value for the [MethodCodec] used.
///
/// This property is `dynamic`, which means type-checking is skipped when accessing
/// this property. To minimize the risk of type errors at runtime, the value should
/// be cast to `Object?` when accessed.
final dynamic arguments;
@override
String toString() => '${objectRuntimeType(this, 'MethodCall')}($method, $arguments)';
}
/// A codec for method calls and enveloped results.
///
/// All operations throw an exception, if conversion fails.
///
/// See also:
///
/// * [MethodChannel], which use [MethodCodec]s for communication
/// between Flutter and platform plugins.
/// * [EventChannel], which use [MethodCodec]s for communication
/// between Flutter and platform plugins.
abstract class MethodCodec {
/// Encodes the specified [methodCall] into binary.
ByteData encodeMethodCall(MethodCall methodCall);
/// Decodes the specified [methodCall] from binary.
MethodCall decodeMethodCall(ByteData? methodCall);
/// Decodes the specified result [envelope] from binary.
///
/// Throws [PlatformException], if [envelope] represents an error, otherwise
/// returns the enveloped result.
///
/// The type returned from [decodeEnvelope] is `dynamic` (not `Object?`),
/// which means *no type checking is performed on its return value*. It is
/// strongly recommended that the return value be immediately cast to a known
/// type to prevent runtime errors due to typos that the type checker could
/// otherwise catch.
dynamic decodeEnvelope(ByteData envelope);
/// Encodes a successful [result] into a binary envelope.
ByteData encodeSuccessEnvelope(Object? result);
/// Encodes an error result into a binary envelope.
///
/// The specified error [code], human-readable error [message] and error
/// [details] correspond to the fields of [PlatformException].
ByteData encodeErrorEnvelope({ required String code, String? message, Object? details});
}
/// Thrown to indicate that a platform interaction failed in the platform
/// plugin.
///
/// See also:
///
/// * [MethodCodec], which throws a [PlatformException], if a received result
/// envelope represents an error.
/// * [MethodChannel.invokeMethod], which completes the returned future
/// with a [PlatformException], if invoking the platform plugin method
/// results in an error envelope.
/// * [EventChannel.receiveBroadcastStream], which emits
/// [PlatformException]s as error events, whenever an event received from the
/// platform plugin is wrapped in an error envelope.
class PlatformException implements Exception {
/// Creates a [PlatformException] with the specified error [code] and optional
/// [message], and with the optional error [details] which must be a valid
/// value for the [MethodCodec] involved in the interaction.
PlatformException({
required this.code,
this.message,
this.details,
this.stacktrace,
});
/// An error code.
final String code;
/// A human-readable error message, possibly null.
final String? message;
/// Error details, possibly null.
///
/// This property is `dynamic`, which means type-checking is skipped when accessing
/// this property. To minimize the risk of type errors at runtime, the value should
/// be cast to `Object?` when accessed.
final dynamic details;
/// Native stacktrace for the error, possibly null.
///
/// This contains the native platform stack trace, not the Dart stack trace.
///
/// The stack trace for Dart exceptions can be obtained using try-catch blocks, for example:
///
/// ```dart
/// try {
/// // ...
/// } catch (e, stacktrace) {
/// print(stacktrace);
/// }
/// ```
///
/// On Android this field is populated when a `RuntimeException` or a subclass of it is thrown in the method call handler,
/// as shown in the following example:
///
/// ```kotlin
/// import androidx.annotation.NonNull
/// import io.flutter.embedding.android.FlutterActivity
/// import io.flutter.embedding.engine.FlutterEngine
/// import io.flutter.plugin.common.MethodChannel
///
/// class MainActivity: FlutterActivity() {
/// private val CHANNEL = "channel_name"
///
/// override fun configureFlutterEngine(@NonNull flutterEngine: FlutterEngine) {
/// super.configureFlutterEngine(flutterEngine)
/// MethodChannel(flutterEngine.dartExecutor.binaryMessenger, CHANNEL).setMethodCallHandler {
/// call, result -> throw RuntimeException("Oh no")
/// }
/// }
/// }
/// ```
///
/// It is also populated on Android if the method channel result is not serializable.
/// If the result is not serializable, an exception gets thrown during the serialization process.
/// This can be seen in the following example:
///
/// ```kotlin
/// import androidx.annotation.NonNull
/// import io.flutter.embedding.android.FlutterActivity
/// import io.flutter.embedding.engine.FlutterEngine
/// import io.flutter.plugin.common.MethodChannel
///
/// class MainActivity: FlutterActivity() {
/// private val CHANNEL = "channel_name"
///
/// override fun configureFlutterEngine(@NonNull flutterEngine: FlutterEngine) {
/// super.configureFlutterEngine(flutterEngine)
/// MethodChannel(flutterEngine.dartExecutor.binaryMessenger, CHANNEL).setMethodCallHandler {
/// call, result -> result.success(Object())
/// }
/// }
/// }
/// ```
///
/// In the cases described above, the content of [stacktrace] will be the unprocessed output of calling `toString()` on the exception.
///
/// MacOS, iOS, Linux and Windows don't support querying the native stacktrace.
///
/// On custom Flutter embedders this value will be null on platforms that don't support querying the call stack.
final String? stacktrace;
@override
String toString() => 'PlatformException($code, $message, $details, $stacktrace)';
}
/// Thrown to indicate that a platform interaction failed to find a handling
/// plugin.
///
/// See also:
///
/// * [MethodChannel.invokeMethod], which completes the returned future
/// with a [MissingPluginException], if no plugin handler for the method call
/// was found.
/// * [OptionalMethodChannel.invokeMethod], which completes the returned future
/// with null, if no plugin handler for the method call was found.
class MissingPluginException implements Exception {
/// Creates a [MissingPluginException] with an optional human-readable
/// error message.
MissingPluginException([this.message]);
/// A human-readable error message, possibly null.
final String? message;
@override
String toString() => 'MissingPluginException($message)';
}
| flutter/packages/flutter/lib/src/services/message_codec.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/message_codec.dart",
"repo_id": "flutter",
"token_count": 2207
} | 667 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
/// Service extension constants for the services library.
///
/// These constants will be used when registering service extensions in the
/// framework, and they will also be used by tools and services that call these
/// service extensions.
///
/// The String value for each of these extension names should be accessed by
/// calling the `.name` property on the enum value.
enum ServicesServiceExtensions {
/// Name of service extension that, when called, will toggle whether
/// statistics about the usage of Platform Channels will be printed out
/// periodically to the console and Timeline events will show the time between
/// sending and receiving a message (encoding and decoding time excluded).
///
/// See also:
///
/// * [debugProfilePlatformChannels], which is the flag that this service
/// extension exposes.
/// * [ServicesBinding.initServiceExtensions], where the service extension is
/// registered.
profilePlatformChannels,
/// Name of service extension that, when called, will evict an image from the
/// rootBundle cache and cause the image cache to be cleared.
///
/// This is used by hot reload mode to clear out the cache of resources that
/// have changed. This service extension should be called with a String value
/// of the image path (e.g. foo.png).
///
/// See also:
///
/// * [ServicesBinding.initServiceExtensions], where the service extension is
/// registered.
evict,
}
| flutter/packages/flutter/lib/src/services/service_extensions.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/services/service_extensions.dart",
"repo_id": "flutter",
"token_count": 390
} | 668 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// The widget in this file is an empty mock for non-web platforms. See
// `_platform_selectable_region_context_menu_web.dart` for the web
// implementation.
import 'framework.dart';
import 'selection_container.dart';
/// Function signature for `ui_web.platformViewRegistry.registerViewFactory`.
@visibleForTesting
typedef RegisterViewFactory = void Function(String, Object Function(int viewId), {bool isVisible});
/// A widget that provides native selection context menu for its child subtree.
///
/// This widget currently only supports Flutter web. Using this widget in non-web
/// platforms will throw [UnimplementedError]s.
///
/// In web platform, this widget registers a singleton platform view, i.e. a
/// HTML DOM element. The created platform view will be shared between all
/// [PlatformSelectableRegionContextMenu]s.
///
/// Only one [SelectionContainerDelegate] can attach to the
/// [PlatformSelectableRegionContextMenu] at a time. Use [attach] method to make
/// a [SelectionContainerDelegate] to be the active client.
class PlatformSelectableRegionContextMenu extends StatelessWidget {
/// Creates a [PlatformSelectableRegionContextMenu]
// ignore: prefer_const_constructors_in_immutables
PlatformSelectableRegionContextMenu({
// ignore: avoid_unused_constructor_parameters
required Widget child,
super.key,
});
/// Attaches the `client` to be able to open platform-appropriate context menus.
static void attach(SelectionContainerDelegate client) => throw UnimplementedError();
/// Detaches the `client` from the platform-appropriate selection context menus.
static void detach(SelectionContainerDelegate client) => throw UnimplementedError();
/// Override this to provide a custom implementation of `ui_web.platformViewRegistry.registerViewFactory`.
///
/// This should only be used for testing.
@visibleForTesting
static RegisterViewFactory? debugOverrideRegisterViewFactory;
@override
Widget build(BuildContext context) => throw UnimplementedError();
}
| flutter/packages/flutter/lib/src/widgets/_platform_selectable_region_context_menu_io.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/_platform_selectable_region_context_menu_io.dart",
"repo_id": "flutter",
"token_count": 565
} | 669 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' as math;
import 'dart:ui' as ui show Image, ImageFilter, TextHeightBehavior;
import 'package:flutter/animation.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'binding.dart';
import 'debug.dart';
import 'framework.dart';
import 'localizations.dart';
import 'visibility.dart';
import 'widget_span.dart';
export 'package:flutter/animation.dart';
export 'package:flutter/foundation.dart' show
ChangeNotifier,
FlutterErrorDetails,
Listenable,
TargetPlatform,
ValueNotifier;
export 'package:flutter/painting.dart';
export 'package:flutter/rendering.dart' show
AlignmentGeometryTween,
AlignmentTween,
Axis,
BoxConstraints,
BoxConstraintsTransform,
CrossAxisAlignment,
CustomClipper,
CustomPainter,
CustomPainterSemantics,
DecorationPosition,
FlexFit,
FlowDelegate,
FlowPaintingContext,
FractionalOffsetTween,
HitTestBehavior,
LayerLink,
MainAxisAlignment,
MainAxisSize,
MouseCursor,
MultiChildLayoutDelegate,
PaintingContext,
PointerCancelEvent,
PointerCancelEventListener,
PointerDownEvent,
PointerDownEventListener,
PointerEvent,
PointerMoveEvent,
PointerMoveEventListener,
PointerUpEvent,
PointerUpEventListener,
RelativeRect,
SemanticsBuilderCallback,
ShaderCallback,
ShapeBorderClipper,
SingleChildLayoutDelegate,
StackFit,
SystemMouseCursors,
TextOverflow,
ValueChanged,
ValueGetter,
WrapAlignment,
WrapCrossAlignment;
export 'package:flutter/services.dart' show
AssetBundle;
// Examples can assume:
// class TestWidget extends StatelessWidget { const TestWidget({super.key}); @override Widget build(BuildContext context) => const Placeholder(); }
// late WidgetTester tester;
// late bool _visible;
// class Sky extends CustomPainter { @override void paint(Canvas c, Size s) {} @override bool shouldRepaint(Sky s) => false; }
// late BuildContext context;
// String userAvatarUrl = '';
// BIDIRECTIONAL TEXT SUPPORT
/// An [InheritedElement] that has hundreds of dependencies but will
/// infrequently change. This provides a performance tradeoff where building
/// the [Widget]s is faster but performing updates is slower.
///
/// | | _UbiquitousInheritedElement | InheritedElement |
/// |---------------------|------------------------------|------------------|
/// | insert (best case) | O(1) | O(1) |
/// | insert (worst case) | O(1) | O(n) |
/// | search (best case) | O(n) | O(1) |
/// | search (worst case) | O(n) | O(n) |
///
/// Insert happens when building the [Widget] tree, search happens when updating
/// [Widget]s.
class _UbiquitousInheritedElement extends InheritedElement {
/// Creates an element that uses the given widget as its configuration.
_UbiquitousInheritedElement(super.widget);
@override
void setDependencies(Element dependent, Object? value) {
// This is where the cost of [InheritedElement] is incurred during build
// time of the widget tree. Omitting this bookkeeping is where the
// performance savings come from.
assert(value == null);
}
@override
Object? getDependencies(Element dependent) {
return null;
}
@override
void notifyClients(InheritedWidget oldWidget) {
_recurseChildren(this, (Element element) {
if (element.doesDependOnInheritedElement(this)) {
notifyDependent(oldWidget, element);
}
});
}
static void _recurseChildren(Element element, ElementVisitor visitor) {
element.visitChildren((Element child) {
_recurseChildren(child, visitor);
});
visitor(element);
}
}
/// See also:
///
/// * [_UbiquitousInheritedElement], the [Element] for [_UbiquitousInheritedWidget].
abstract class _UbiquitousInheritedWidget extends InheritedWidget {
const _UbiquitousInheritedWidget({super.key, required super.child});
@override
InheritedElement createElement() => _UbiquitousInheritedElement(this);
}
/// A widget that determines the ambient directionality of text and
/// text-direction-sensitive render objects.
///
/// For example, [Padding] depends on the [Directionality] to resolve
/// [EdgeInsetsDirectional] objects into absolute [EdgeInsets] objects.
class Directionality extends _UbiquitousInheritedWidget {
/// Creates a widget that determines the directionality of text and
/// text-direction-sensitive render objects.
const Directionality({
super.key,
required this.textDirection,
required super.child,
});
/// The text direction for this subtree.
final TextDirection textDirection;
/// The text direction from the closest instance of this class that encloses
/// the given context.
///
/// If there is no [Directionality] ancestor widget in the tree at the given
/// context, then this will throw a descriptive [FlutterError] in debug mode
/// and an exception in release mode.
///
/// Typical usage is as follows:
///
/// ```dart
/// TextDirection textDirection = Directionality.of(context);
/// ```
///
/// See also:
///
/// * [maybeOf], which will return null if no [Directionality] ancestor
/// widget is in the tree.
static TextDirection of(BuildContext context) {
assert(debugCheckHasDirectionality(context));
final Directionality widget = context.dependOnInheritedWidgetOfExactType<Directionality>()!;
return widget.textDirection;
}
/// The text direction from the closest instance of this class that encloses
/// the given context.
///
/// If there is no [Directionality] ancestor widget in the tree at the given
/// context, then this will return null.
///
/// Typical usage is as follows:
///
/// ```dart
/// TextDirection? textDirection = Directionality.maybeOf(context);
/// ```
///
/// See also:
///
/// * [of], which will throw if no [Directionality] ancestor widget is in the
/// tree.
static TextDirection? maybeOf(BuildContext context) {
final Directionality? widget = context.dependOnInheritedWidgetOfExactType<Directionality>();
return widget?.textDirection;
}
@override
bool updateShouldNotify(Directionality oldWidget) => textDirection != oldWidget.textDirection;
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<TextDirection>('textDirection', textDirection));
}
}
// PAINTING NODES
/// A widget that makes its child partially transparent.
///
/// This class paints its child into an intermediate buffer and then blends the
/// child back into the scene partially transparent.
///
/// For values of opacity other than 0.0 and 1.0, this class is relatively
/// expensive because it requires painting the child into an intermediate
/// buffer. For the value 0.0, the child is not painted at all. For the
/// value 1.0, the child is painted immediately without an intermediate buffer.
///
/// The presence of the intermediate buffer which has a transparent background
/// by default may cause some child widgets to behave differently. For example
/// a [BackdropFilter] child will only be able to apply its filter to the content
/// between this widget and the backdrop child and may require adjusting the
/// [BackdropFilter.blendMode] property to produce the desired results.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=9hltevOHQBw}
///
/// {@tool snippet}
///
/// This example shows some [Text] when the `_visible` member field is true, and
/// hides it when it is false:
///
/// ```dart
/// Opacity(
/// opacity: _visible ? 1.0 : 0.0,
/// child: const Text("Now you see me, now you don't!"),
/// )
/// ```
/// {@end-tool}
///
/// This is more efficient than adding and removing the child widget from the
/// tree on demand.
///
/// ## Performance considerations for opacity animation
///
/// Animating an [Opacity] widget directly causes the widget (and possibly its
/// subtree) to rebuild each frame, which is not very efficient. Consider using
/// one of these alternative widgets instead:
///
/// * [AnimatedOpacity], which uses an animation internally to efficiently
/// animate opacity.
/// * [FadeTransition], which uses a provided animation to efficiently animate
/// opacity.
///
/// ## Transparent image
///
/// If only a single [Image] or [Color] needs to be composited with an opacity
/// between 0.0 and 1.0, it's much faster to directly use them without [Opacity]
/// widgets.
///
/// For example, `Container(color: Color.fromRGBO(255, 0, 0, 0.5))` is much
/// faster than `Opacity(opacity: 0.5, child: Container(color: Colors.red))`.
///
/// {@tool snippet}
///
/// The following example draws an [Image] with 0.5 opacity without using
/// [Opacity]:
///
/// ```dart
/// Image.network(
/// 'https://raw.githubusercontent.com/flutter/assets-for-api-docs/main/packages/diagrams/assets/blend_mode_destination.jpeg',
/// color: const Color.fromRGBO(255, 255, 255, 0.5),
/// colorBlendMode: BlendMode.modulate
/// )
/// ```
///
/// {@end-tool}
///
/// Directly drawing an [Image] or [Color] with opacity is faster than using
/// [Opacity] on top of them because [Opacity] could apply the opacity to a
/// group of widgets and therefore a costly offscreen buffer will be used.
/// Drawing content into the offscreen buffer may also trigger render target
/// switches and such switching is particularly slow in older GPUs.
///
/// ## Hit testing
///
/// Setting the [opacity] to zero does not prevent hit testing from being applied
/// to the descendants of the [Opacity] widget. This can be confusing for the
/// user, who may not see anything, and may believe the area of the interface
/// where the [Opacity] is hiding a widget to be non-interactive.
///
/// With certain widgets, such as [Flow], that compute their positions only when
/// they are painted, this can actually lead to bugs (from unexpected geometry
/// to exceptions), because those widgets are not painted by the [Opacity]
/// widget at all when the [opacity] is zero.
///
/// To avoid such problems, it is generally a good idea to use an
/// [IgnorePointer] widget when setting the [opacity] to zero. This prevents
/// interactions with any children in the subtree.
///
/// See also:
///
/// * [Visibility], which can hide a child more efficiently (albeit less
/// subtly, because it is either visible or hidden, rather than allowing
/// fractional opacity values). Specifically, the [Visibility.maintain]
/// constructor is equivalent to using an opacity widget with values of
/// `0.0` or `1.0`.
/// * [ShaderMask], which can apply more elaborate effects to its child.
/// * [Transform], which applies an arbitrary transform to its child widget at
/// paint time.
/// * [SliverOpacity], the sliver version of this widget.
class Opacity extends SingleChildRenderObjectWidget {
/// Creates a widget that makes its child partially transparent.
///
/// The [opacity] argument must be between zero and one, inclusive.
const Opacity({
super.key,
required this.opacity,
this.alwaysIncludeSemantics = false,
super.child,
}) : assert(opacity >= 0.0 && opacity <= 1.0);
/// The fraction to scale the child's alpha value.
///
/// An opacity of one is fully opaque. An opacity of zero is fully transparent
/// (i.e., invisible).
///
/// Values one and zero are painted with a fast path. Other values require
/// painting the child into an intermediate buffer, which is expensive.
final double opacity;
/// Whether the semantic information of the children is always included.
///
/// Defaults to false.
///
/// When true, regardless of the opacity settings the child semantic
/// information is exposed as if the widget were fully visible. This is
/// useful in cases where labels may be hidden during animations that
/// would otherwise contribute relevant semantics.
final bool alwaysIncludeSemantics;
@override
RenderOpacity createRenderObject(BuildContext context) {
return RenderOpacity(
opacity: opacity,
alwaysIncludeSemantics: alwaysIncludeSemantics,
);
}
@override
void updateRenderObject(BuildContext context, RenderOpacity renderObject) {
renderObject
..opacity = opacity
..alwaysIncludeSemantics = alwaysIncludeSemantics;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('opacity', opacity));
properties.add(FlagProperty('alwaysIncludeSemantics', value: alwaysIncludeSemantics, ifTrue: 'alwaysIncludeSemantics'));
}
}
/// A widget that applies a mask generated by a [Shader] to its child.
///
/// For example, [ShaderMask] can be used to gradually fade out the edge
/// of a child by using a [ui.Gradient.linear] mask.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=7sUL66pTQ7Q}
///
/// {@tool snippet}
///
/// This example makes the text look like it is on fire:
///
/// ```dart
/// ShaderMask(
/// shaderCallback: (Rect bounds) {
/// return RadialGradient(
/// center: Alignment.topLeft,
/// radius: 1.0,
/// colors: <Color>[Colors.yellow, Colors.deepOrange.shade900],
/// tileMode: TileMode.mirror,
/// ).createShader(bounds);
/// },
/// child: const Text(
/// "I'm burning the memories",
/// style: TextStyle(color: Colors.white),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [Opacity], which can apply a uniform alpha effect to its child.
/// * [CustomPaint], which lets you draw directly on the canvas.
/// * [DecoratedBox], for another approach at decorating child widgets.
/// * [BackdropFilter], which applies an image filter to the background.
class ShaderMask extends SingleChildRenderObjectWidget {
/// Creates a widget that applies a mask generated by a [Shader] to its child.
const ShaderMask({
super.key,
required this.shaderCallback,
this.blendMode = BlendMode.modulate,
super.child,
});
/// Called to create the [dart:ui.Shader] that generates the mask.
///
/// The shader callback is called with the current size of the child so that
/// it can customize the shader to the size and location of the child.
///
/// Typically this will use a [LinearGradient], [RadialGradient], or
/// [SweepGradient] to create the [dart:ui.Shader], though the
/// [dart:ui.ImageShader] class could also be used.
final ShaderCallback shaderCallback;
/// The [BlendMode] to use when applying the shader to the child.
///
/// The default, [BlendMode.modulate], is useful for applying an alpha blend
/// to the child. Other blend modes can be used to create other effects.
final BlendMode blendMode;
@override
RenderShaderMask createRenderObject(BuildContext context) {
return RenderShaderMask(
shaderCallback: shaderCallback,
blendMode: blendMode,
);
}
@override
void updateRenderObject(BuildContext context, RenderShaderMask renderObject) {
renderObject
..shaderCallback = shaderCallback
..blendMode = blendMode;
}
}
/// A widget that applies a filter to the existing painted content and then
/// paints [child].
///
/// The filter will be applied to all the area within its parent or ancestor
/// widget's clip. If there's no clip, the filter will be applied to the full
/// screen.
///
/// The results of the filter will be blended back into the background using
/// the [blendMode] parameter.
/// {@template flutter.widgets.BackdropFilter.blendMode}
/// The only value for [blendMode] that is supported on all platforms is
/// [BlendMode.srcOver] which works well for most scenes. But that value may
/// produce surprising results when a parent of the [BackdropFilter] uses a
/// temporary buffer, or save layer, as does an [Opacity] widget. In that
/// situation, a value of [BlendMode.src] can produce more pleasing results,
/// but at the cost of incompatibility with some platforms, most notably the
/// html renderer for web applications.
/// {@endtemplate}
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=dYRs7Q1vfYI}
///
/// {@tool snippet}
/// If the [BackdropFilter] needs to be applied to an area that exactly matches
/// its child, wraps the [BackdropFilter] with a clip widget that clips exactly
/// to that child.
///
/// ```dart
/// Stack(
/// fit: StackFit.expand,
/// children: <Widget>[
/// Text('0' * 10000),
/// Center(
/// child: ClipRect( // <-- clips to the 200x200 [Container] below
/// child: BackdropFilter(
/// filter: ui.ImageFilter.blur(
/// sigmaX: 5.0,
/// sigmaY: 5.0,
/// ),
/// child: Container(
/// alignment: Alignment.center,
/// width: 200.0,
/// height: 200.0,
/// child: const Text('Hello World'),
/// ),
/// ),
/// ),
/// ),
/// ],
/// )
/// ```
/// {@end-tool}
///
/// This effect is relatively expensive, especially if the filter is non-local,
/// such as a blur.
///
/// If all you want to do is apply an [ImageFilter] to a single widget
/// (as opposed to applying the filter to everything _beneath_ a widget), use
/// [ImageFiltered] instead. For that scenario, [ImageFiltered] is both
/// easier to use and less expensive than [BackdropFilter].
///
/// {@tool snippet}
///
/// This example shows how the common case of applying a [BackdropFilter] blur
/// to a single sibling can be replaced with an [ImageFiltered] widget. This code
/// is generally simpler and the performance will be improved dramatically for
/// complex filters like blurs.
///
/// The implementation below is unnecessarily expensive.
///
/// ```dart
/// Widget buildBackdrop() {
/// return Stack(
/// children: <Widget>[
/// Positioned.fill(child: Image.asset('image.png')),
/// Positioned.fill(
/// child: BackdropFilter(
/// filter: ui.ImageFilter.blur(sigmaX: 6, sigmaY: 6),
/// ),
/// ),
/// ],
/// );
/// }
/// ```
/// {@end-tool}
/// {@tool snippet}
/// Instead consider the following approach which directly applies a blur
/// to the child widget.
///
/// ```dart
/// Widget buildFilter() {
/// return ImageFiltered(
/// imageFilter: ui.ImageFilter.blur(sigmaX: 6, sigmaY: 6),
/// child: Image.asset('image.png'),
/// );
/// }
/// ```
///
/// {@end-tool}
///
/// See also:
///
/// * [ImageFiltered], which applies an [ImageFilter] to its child.
/// * [DecoratedBox], which draws a background under (or over) a widget.
/// * [Opacity], which changes the opacity of the widget itself.
/// * https://flutter.dev/go/ios-platformview-backdrop-filter-blur for details and restrictions when an iOS PlatformView needs to be blurred.
class BackdropFilter extends SingleChildRenderObjectWidget {
/// Creates a backdrop filter.
///
/// The [blendMode] argument will default to [BlendMode.srcOver] and must not be
/// null if provided.
const BackdropFilter({
super.key,
required this.filter,
super.child,
this.blendMode = BlendMode.srcOver,
});
/// The image filter to apply to the existing painted content before painting the child.
///
/// For example, consider using [ImageFilter.blur] to create a backdrop
/// blur effect.
final ui.ImageFilter filter;
/// The blend mode to use to apply the filtered background content onto the background
/// surface.
///
/// {@macro flutter.widgets.BackdropFilter.blendMode}
final BlendMode blendMode;
@override
RenderBackdropFilter createRenderObject(BuildContext context) {
return RenderBackdropFilter(filter: filter, blendMode: blendMode);
}
@override
void updateRenderObject(BuildContext context, RenderBackdropFilter renderObject) {
renderObject
..filter = filter
..blendMode = blendMode;
}
}
/// A widget that provides a canvas on which to draw during the paint phase.
///
/// When asked to paint, [CustomPaint] first asks its [painter] to paint on the
/// current canvas, then it paints its child, and then, after painting its
/// child, it asks its [foregroundPainter] to paint. The coordinate system of the
/// canvas matches the coordinate system of the [CustomPaint] object. The
/// painters are expected to paint within a rectangle starting at the origin and
/// encompassing a region of the given size. (If the painters paint outside
/// those bounds, there might be insufficient memory allocated to rasterize the
/// painting commands and the resulting behavior is undefined.) To enforce
/// painting within those bounds, consider wrapping this [CustomPaint] with a
/// [ClipRect] widget.
///
/// Painters are implemented by subclassing [CustomPainter].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=kp14Y4uHpHs}
///
/// Because custom paint calls its painters during paint, you cannot call
/// `setState` or `markNeedsLayout` during the callback (the layout for this
/// frame has already happened).
///
/// Custom painters normally size themselves to their [child]. If they do not
/// have a child, they attempt to size themselves to the specified [size], which
/// defaults to [Size.zero]. The parent [may enforce constraints on this
/// size](https://docs.flutter.dev/ui/layout/constraints).
///
/// The [isComplex] and [willChange] properties are hints to the compositor's
/// raster cache.
///
/// {@tool snippet}
///
/// This example shows how the sample custom painter shown at [CustomPainter]
/// could be used in a [CustomPaint] widget to display a background to some
/// text.
///
/// ```dart
/// CustomPaint(
/// painter: Sky(),
/// child: const Center(
/// child: Text(
/// 'Once upon a time...',
/// style: TextStyle(
/// fontSize: 40.0,
/// fontWeight: FontWeight.w900,
/// color: Color(0xFFFFFFFF),
/// ),
/// ),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [CustomPainter], the class to extend when creating custom painters.
/// * [Canvas], the class that a custom painter uses to paint.
class CustomPaint extends SingleChildRenderObjectWidget {
/// Creates a widget that delegates its painting.
const CustomPaint({
super.key,
this.painter,
this.foregroundPainter,
this.size = Size.zero,
this.isComplex = false,
this.willChange = false,
super.child,
}) : assert(painter != null || foregroundPainter != null || (!isComplex && !willChange));
/// The painter that paints before the children.
final CustomPainter? painter;
/// The painter that paints after the children.
final CustomPainter? foregroundPainter;
/// The size that this [CustomPaint] should aim for, given the layout
/// constraints, if there is no child.
///
/// Defaults to [Size.zero].
///
/// If there's a child, this is ignored, and the size of the child is used
/// instead.
final Size size;
/// Whether the painting is complex enough to benefit from caching.
///
/// The compositor contains a raster cache that holds bitmaps of layers in
/// order to avoid the cost of repeatedly rendering those layers on each
/// frame. If this flag is not set, then the compositor will apply its own
/// heuristics to decide whether the layer containing this widget is complex
/// enough to benefit from caching.
///
/// This flag can't be set to true if both [painter] and [foregroundPainter]
/// are null because this flag will be ignored in such case.
final bool isComplex;
/// Whether the raster cache should be told that this painting is likely
/// to change in the next frame.
///
/// This hint tells the compositor not to cache the layer containing this
/// widget because the cache will not be used in the future. If this hint is
/// not set, the compositor will apply its own heuristics to decide whether
/// the layer is likely to be reused in the future.
///
/// This flag can't be set to true if both [painter] and [foregroundPainter]
/// are null because this flag will be ignored in such case.
final bool willChange;
@override
RenderCustomPaint createRenderObject(BuildContext context) {
return RenderCustomPaint(
painter: painter,
foregroundPainter: foregroundPainter,
preferredSize: size,
isComplex: isComplex,
willChange: willChange,
);
}
@override
void updateRenderObject(BuildContext context, RenderCustomPaint renderObject) {
renderObject
..painter = painter
..foregroundPainter = foregroundPainter
..preferredSize = size
..isComplex = isComplex
..willChange = willChange;
}
@override
void didUnmountRenderObject(RenderCustomPaint renderObject) {
renderObject
..painter = null
..foregroundPainter = null;
}
}
/// A widget that clips its child using a rectangle.
///
/// By default, [ClipRect] prevents its child from painting outside its
/// bounds, but the size and location of the clip rect can be customized using a
/// custom [clipper].
///
/// [ClipRect] is commonly used with these widgets, which commonly paint outside
/// their bounds:
///
/// * [CustomPaint]
/// * [CustomSingleChildLayout]
/// * [CustomMultiChildLayout]
/// * [Align] and [Center] (e.g., if [Align.widthFactor] or
/// [Align.heightFactor] is less than 1.0).
/// * [OverflowBox]
/// * [SizedOverflowBox]
///
/// {@tool snippet}
///
/// For example, by combining a [ClipRect] with an [Align], one can show just
/// the top half of an [Image]:
///
/// ```dart
/// ClipRect(
/// child: Align(
/// alignment: Alignment.topCenter,
/// heightFactor: 0.5,
/// child: Image.network(userAvatarUrl),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [CustomClipper], for information about creating custom clips.
/// * [ClipRRect], for a clip with rounded corners.
/// * [ClipOval], for an elliptical clip.
/// * [ClipPath], for an arbitrarily shaped clip.
class ClipRect extends SingleChildRenderObjectWidget {
/// Creates a rectangular clip.
///
/// If [clipper] is null, the clip will match the layout size and position of
/// the child.
///
/// If [clipBehavior] is [Clip.none], no clipping will be applied.
const ClipRect({
super.key,
this.clipper,
this.clipBehavior = Clip.hardEdge,
super.child,
});
/// If non-null, determines which clip to use.
final CustomClipper<Rect>? clipper;
/// {@macro flutter.rendering.ClipRectLayer.clipBehavior}
///
/// Defaults to [Clip.hardEdge].
final Clip clipBehavior;
@override
RenderClipRect createRenderObject(BuildContext context) {
return RenderClipRect(clipper: clipper, clipBehavior: clipBehavior);
}
@override
void updateRenderObject(BuildContext context, RenderClipRect renderObject) {
renderObject
..clipper = clipper
..clipBehavior = clipBehavior;
}
@override
void didUnmountRenderObject(RenderClipRect renderObject) {
renderObject.clipper = null;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<CustomClipper<Rect>>('clipper', clipper, defaultValue: null));
}
}
/// A widget that clips its child using a rounded rectangle.
///
/// By default, [ClipRRect] uses its own bounds as the base rectangle for the
/// clip, but the size and location of the clip can be customized using a custom
/// [clipper].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=eI43jkQkrvs}
///
/// {@tool dartpad}
/// This example shows various [ClipRRect]s applied to containers.
///
/// ** See code in examples/api/lib/widgets/basic/clip_rrect.0.dart **
/// {@end-tool}
///
/// ## Troubleshooting
///
/// ### Why doesn't my [ClipRRect] child have rounded corners?
///
/// When a [ClipRRect] is bigger than the child it contains, its rounded corners
/// could be drawn in unexpected positions. Make sure that [ClipRRect] and its child
/// have the same bounds (by shrinking the [ClipRRect] with a [FittedBox] or by
/// growing the child).
///
/// {@tool dartpad}
/// This example shows a [ClipRRect] that adds round corners to an image.
///
/// ** See code in examples/api/lib/widgets/basic/clip_rrect.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [CustomClipper], for information about creating custom clips.
/// * [ClipRect], for more efficient clips without rounded corners.
/// * [ClipOval], for an elliptical clip.
/// * [ClipPath], for an arbitrarily shaped clip.
class ClipRRect extends SingleChildRenderObjectWidget {
/// Creates a rounded-rectangular clip.
///
/// The [borderRadius] defaults to [BorderRadius.zero], i.e. a rectangle with
/// right-angled corners.
///
/// If [clipper] is non-null, then [borderRadius] is ignored.
///
/// If [clipBehavior] is [Clip.none], no clipping will be applied.
const ClipRRect({
super.key,
this.borderRadius = BorderRadius.zero,
this.clipper,
this.clipBehavior = Clip.antiAlias,
super.child,
});
/// The border radius of the rounded corners.
///
/// Values are clamped so that horizontal and vertical radii sums do not
/// exceed width/height.
///
/// This value is ignored if [clipper] is non-null.
final BorderRadiusGeometry borderRadius;
/// If non-null, determines which clip to use.
final CustomClipper<RRect>? clipper;
/// {@macro flutter.rendering.ClipRectLayer.clipBehavior}
///
/// Defaults to [Clip.antiAlias].
final Clip clipBehavior;
@override
RenderClipRRect createRenderObject(BuildContext context) {
return RenderClipRRect(
borderRadius: borderRadius,
clipper: clipper,
clipBehavior: clipBehavior,
textDirection: Directionality.maybeOf(context),
);
}
@override
void updateRenderObject(BuildContext context, RenderClipRRect renderObject) {
renderObject
..borderRadius = borderRadius
..clipBehavior = clipBehavior
..clipper = clipper
..textDirection = Directionality.maybeOf(context);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<BorderRadiusGeometry>('borderRadius', borderRadius, showName: false, defaultValue: null));
properties.add(DiagnosticsProperty<CustomClipper<RRect>>('clipper', clipper, defaultValue: null));
}
}
/// A widget that clips its child using an oval.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=vzWWDO6whIM}
///
/// By default, inscribes an axis-aligned oval into its layout dimensions and
/// prevents its child from painting outside that oval, but the size and
/// location of the clip oval can be customized using a custom [clipper].
///
/// See also:
///
/// * [CustomClipper], for information about creating custom clips.
/// * [ClipRect], for more efficient clips without rounded corners.
/// * [ClipRRect], for a clip with rounded corners.
/// * [ClipPath], for an arbitrarily shaped clip.
class ClipOval extends SingleChildRenderObjectWidget {
/// Creates an oval-shaped clip.
///
/// If [clipper] is null, the oval will be inscribed into the layout size and
/// position of the child.
///
/// If [clipBehavior] is [Clip.none], no clipping will be applied.
const ClipOval({
super.key,
this.clipper,
this.clipBehavior = Clip.antiAlias,
super.child,
});
/// If non-null, determines which clip to use.
///
/// The delegate returns a rectangle that describes the axis-aligned
/// bounding box of the oval. The oval's axes will themselves also
/// be axis-aligned.
///
/// If the [clipper] delegate is null, then the oval uses the
/// widget's bounding box (the layout dimensions of the render
/// object) instead.
final CustomClipper<Rect>? clipper;
/// {@macro flutter.rendering.ClipRectLayer.clipBehavior}
///
/// Defaults to [Clip.antiAlias].
final Clip clipBehavior;
@override
RenderClipOval createRenderObject(BuildContext context) {
return RenderClipOval(clipper: clipper, clipBehavior: clipBehavior);
}
@override
void updateRenderObject(BuildContext context, RenderClipOval renderObject) {
renderObject
..clipper = clipper
..clipBehavior = clipBehavior;
}
@override
void didUnmountRenderObject(RenderClipOval renderObject) {
renderObject.clipper = null;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<CustomClipper<Rect>>('clipper', clipper, defaultValue: null));
}
}
/// A widget that clips its child using a path.
///
/// Calls a callback on a delegate whenever the widget is to be
/// painted. The callback returns a path and the widget prevents the
/// child from painting outside the path.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=oAUebVIb-7s}
///
/// Clipping to a path is expensive. Certain shapes have more
/// optimized widgets:
///
/// * To clip to a rectangle, consider [ClipRect].
/// * To clip to an oval or circle, consider [ClipOval].
/// * To clip to a rounded rectangle, consider [ClipRRect].
///
/// To clip to a particular [ShapeBorder], consider using either the
/// [ClipPath.shape] static method or the [ShapeBorderClipper] custom clipper
/// class.
class ClipPath extends SingleChildRenderObjectWidget {
/// Creates a path clip.
///
/// If [clipper] is null, the clip will be a rectangle that matches the layout
/// size and location of the child. However, rather than use this default,
/// consider using a [ClipRect], which can achieve the same effect more
/// efficiently.
///
/// If [clipBehavior] is [Clip.none], no clipping will be applied.
const ClipPath({
super.key,
this.clipper,
this.clipBehavior = Clip.antiAlias,
super.child,
});
/// Creates a shape clip.
///
/// Uses a [ShapeBorderClipper] to configure the [ClipPath] to clip to the
/// given [ShapeBorder].
static Widget shape({
Key? key,
required ShapeBorder shape,
Clip clipBehavior = Clip.antiAlias,
Widget? child,
}) {
return Builder(
key: key,
builder: (BuildContext context) {
return ClipPath(
clipper: ShapeBorderClipper(
shape: shape,
textDirection: Directionality.maybeOf(context),
),
clipBehavior: clipBehavior,
child: child,
);
},
);
}
/// If non-null, determines which clip to use.
///
/// The default clip, which is used if this property is null, is the
/// bounding box rectangle of the widget. [ClipRect] is a more
/// efficient way of obtaining that effect.
final CustomClipper<Path>? clipper;
/// {@macro flutter.rendering.ClipRectLayer.clipBehavior}
///
/// Defaults to [Clip.antiAlias].
final Clip clipBehavior;
@override
RenderClipPath createRenderObject(BuildContext context) {
return RenderClipPath(clipper: clipper, clipBehavior: clipBehavior);
}
@override
void updateRenderObject(BuildContext context, RenderClipPath renderObject) {
renderObject
..clipper = clipper
..clipBehavior = clipBehavior;
}
@override
void didUnmountRenderObject(RenderClipPath renderObject) {
renderObject.clipper = null;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<CustomClipper<Path>>('clipper', clipper, defaultValue: null));
}
}
/// A widget representing a physical layer that clips its children to a shape.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=XgUOSS30OQk}
///
/// Physical layers cast shadows based on an [elevation] which is nominally in
/// logical pixels, coming vertically out of the rendering surface.
///
/// For shapes that cannot be expressed as a rectangle with rounded corners use
/// [PhysicalShape].
///
/// See also:
///
/// * [AnimatedPhysicalModel], which animates property changes smoothly over
/// a given duration.
/// * [DecoratedBox], which can apply more arbitrary shadow effects.
/// * [ClipRect], which applies a clip to its child.
class PhysicalModel extends SingleChildRenderObjectWidget {
/// Creates a physical model with a rounded-rectangular clip.
///
/// The [color] is required; physical things have a color.
///
/// The [shape], [elevation], [color], [clipBehavior], and [shadowColor] must
/// not be null. Additionally, the [elevation] must be non-negative.
const PhysicalModel({
super.key,
this.shape = BoxShape.rectangle,
this.clipBehavior = Clip.none,
this.borderRadius,
this.elevation = 0.0,
required this.color,
this.shadowColor = const Color(0xFF000000),
super.child,
}) : assert(elevation >= 0.0);
/// The type of shape.
final BoxShape shape;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.none].
final Clip clipBehavior;
/// The border radius of the rounded corners.
///
/// Values are clamped so that horizontal and vertical radii sums do not
/// exceed width/height.
///
/// This is ignored if the [shape] is not [BoxShape.rectangle].
final BorderRadius? borderRadius;
/// The z-coordinate relative to the parent at which to place this physical
/// object.
///
/// The value is non-negative.
final double elevation;
/// The background color.
final Color color;
/// The shadow color.
final Color shadowColor;
@override
RenderPhysicalModel createRenderObject(BuildContext context) {
return RenderPhysicalModel(
shape: shape,
clipBehavior: clipBehavior,
borderRadius: borderRadius,
elevation: elevation,
color: color,
shadowColor: shadowColor,
);
}
@override
void updateRenderObject(BuildContext context, RenderPhysicalModel renderObject) {
renderObject
..shape = shape
..clipBehavior = clipBehavior
..borderRadius = borderRadius
..elevation = elevation
..color = color
..shadowColor = shadowColor;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<BoxShape>('shape', shape));
properties.add(DiagnosticsProperty<BorderRadius>('borderRadius', borderRadius));
properties.add(DoubleProperty('elevation', elevation));
properties.add(ColorProperty('color', color));
properties.add(ColorProperty('shadowColor', shadowColor));
}
}
/// A widget representing a physical layer that clips its children to a path.
///
/// Physical layers cast shadows based on an [elevation] which is nominally in
/// logical pixels, coming vertically out of the rendering surface.
///
/// [PhysicalModel] does the same but only supports shapes that can be expressed
/// as rectangles with rounded corners.
///
/// {@tool dartpad}
/// This example shows how to use a [PhysicalShape] on a centered [SizedBox]
/// to clip it to a rounded rectangle using a [ShapeBorderClipper] and give it
/// an orange color along with a shadow.
///
/// ** See code in examples/api/lib/widgets/basic/physical_shape.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [ShapeBorderClipper], which converts a [ShapeBorder] to a [CustomClipper], as
/// needed by this widget.
class PhysicalShape extends SingleChildRenderObjectWidget {
/// Creates a physical model with an arbitrary shape clip.
///
/// The [color] is required; physical things have a color.
///
/// The [elevation] must be non-negative.
const PhysicalShape({
super.key,
required this.clipper,
this.clipBehavior = Clip.none,
this.elevation = 0.0,
required this.color,
this.shadowColor = const Color(0xFF000000),
super.child,
}) : assert(elevation >= 0.0);
/// Determines which clip to use.
///
/// If the path in question is expressed as a [ShapeBorder] subclass,
/// consider using the [ShapeBorderClipper] delegate class to adapt the
/// shape for use with this widget.
final CustomClipper<Path> clipper;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.none].
final Clip clipBehavior;
/// The z-coordinate relative to the parent at which to place this physical
/// object.
///
/// The value is non-negative.
final double elevation;
/// The background color.
final Color color;
/// When elevation is non zero the color to use for the shadow color.
final Color shadowColor;
@override
RenderPhysicalShape createRenderObject(BuildContext context) {
return RenderPhysicalShape(
clipper: clipper,
clipBehavior: clipBehavior,
elevation: elevation,
color: color,
shadowColor: shadowColor,
);
}
@override
void updateRenderObject(BuildContext context, RenderPhysicalShape renderObject) {
renderObject
..clipper = clipper
..clipBehavior = clipBehavior
..elevation = elevation
..color = color
..shadowColor = shadowColor;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<CustomClipper<Path>>('clipper', clipper));
properties.add(DoubleProperty('elevation', elevation));
properties.add(ColorProperty('color', color));
properties.add(ColorProperty('shadowColor', shadowColor));
}
}
// POSITIONING AND SIZING NODES
/// A widget that applies a transformation before painting its child.
///
/// Unlike [RotatedBox], which applies a rotation prior to layout, this object
/// applies its transformation just prior to painting, which means the
/// transformation is not taken into account when calculating how much space
/// this widget's child (and thus this widget) consumes.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=9z_YNlRlWfA}
///
/// {@tool snippet}
///
/// This example rotates and skews an orange box containing text, keeping the
/// top right corner pinned to its original position.
///
/// ```dart
/// ColoredBox(
/// color: Colors.black,
/// child: Transform(
/// alignment: Alignment.topRight,
/// transform: Matrix4.skewY(0.3)..rotateZ(-math.pi / 12.0),
/// child: Container(
/// padding: const EdgeInsets.all(8.0),
/// color: const Color(0xFFE8581C),
/// child: const Text('Apartment for rent!'),
/// ),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [RotatedBox], which rotates the child widget during layout, not just
/// during painting.
/// * [FractionalTranslation], which applies a translation to the child
/// that is relative to the child's size.
/// * [FittedBox], which sizes and positions its child widget to fit the parent
/// according to a given [BoxFit] discipline.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class Transform extends SingleChildRenderObjectWidget {
/// Creates a widget that transforms its child.
const Transform({
super.key,
required this.transform,
this.origin,
this.alignment,
this.transformHitTests = true,
this.filterQuality,
super.child,
});
/// Creates a widget that transforms its child using a rotation around the
/// center.
///
/// The `angle` argument gives the rotation in clockwise radians.
///
/// {@tool snippet}
///
/// This example rotates an orange box containing text around its center by
/// fifteen degrees.
///
/// ```dart
/// Transform.rotate(
/// angle: -math.pi / 12.0,
/// child: Container(
/// padding: const EdgeInsets.all(8.0),
/// color: const Color(0xFFE8581C),
/// child: const Text('Apartment for rent!'),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [RotationTransition], which animates changes in rotation smoothly
/// over a given duration.
Transform.rotate({
super.key,
required double angle,
this.origin,
this.alignment = Alignment.center,
this.transformHitTests = true,
this.filterQuality,
super.child,
}) : transform = _computeRotation(angle);
/// Creates a widget that transforms its child using a translation.
///
/// The `offset` argument specifies the translation.
///
/// {@tool snippet}
///
/// This example shifts the silver-colored child down by fifteen pixels.
///
/// ```dart
/// Transform.translate(
/// offset: const Offset(0.0, 15.0),
/// child: Container(
/// padding: const EdgeInsets.all(8.0),
/// color: const Color(0xFF7F7F7F),
/// child: const Text('Quarter'),
/// ),
/// )
/// ```
/// {@end-tool}
Transform.translate({
super.key,
required Offset offset,
this.transformHitTests = true,
this.filterQuality,
super.child,
}) : transform = Matrix4.translationValues(offset.dx, offset.dy, 0.0),
origin = null,
alignment = null;
/// Creates a widget that scales its child along the 2D plane.
///
/// The `scaleX` argument provides the scalar by which to multiply the `x`
/// axis, and the `scaleY` argument provides the scalar by which to multiply
/// the `y` axis. Either may be omitted, in which case the scaling factor for
/// that axis defaults to 1.0.
///
/// For convenience, to scale the child uniformly, instead of providing
/// `scaleX` and `scaleY`, the `scale` parameter may be used.
///
/// At least one of `scale`, `scaleX`, and `scaleY` must be non-null. If
/// `scale` is provided, the other two must be null; similarly, if it is not
/// provided, one of the other two must be provided.
///
/// The [alignment] controls the origin of the scale; by default, this is the
/// center of the box.
///
/// {@tool snippet}
///
/// This example shrinks an orange box containing text such that each
/// dimension is half the size it would otherwise be.
///
/// ```dart
/// Transform.scale(
/// scale: 0.5,
/// child: Container(
/// padding: const EdgeInsets.all(8.0),
/// color: const Color(0xFFE8581C),
/// child: const Text('Bad Idea Bears'),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [ScaleTransition], which animates changes in scale smoothly over a given
/// duration.
Transform.scale({
super.key,
double? scale,
double? scaleX,
double? scaleY,
this.origin,
this.alignment = Alignment.center,
this.transformHitTests = true,
this.filterQuality,
super.child,
}) : assert(!(scale == null && scaleX == null && scaleY == null), "At least one of 'scale', 'scaleX' and 'scaleY' is required to be non-null"),
assert(scale == null || (scaleX == null && scaleY == null), "If 'scale' is non-null then 'scaleX' and 'scaleY' must be left null"),
transform = Matrix4.diagonal3Values(scale ?? scaleX ?? 1.0, scale ?? scaleY ?? 1.0, 1.0);
/// Creates a widget that mirrors its child about the widget's center point.
///
/// If `flipX` is true, the child widget will be flipped horizontally. Defaults to false.
///
/// If `flipY` is true, the child widget will be flipped vertically. Defaults to false.
///
/// If both are true, the child widget will be flipped both vertically and horizontally, equivalent to a 180 degree rotation.
///
/// {@tool snippet}
///
/// This example flips the text horizontally.
///
/// ```dart
/// Transform.flip(
/// flipX: true,
/// child: const Text('Horizontal Flip'),
/// )
/// ```
/// {@end-tool}
Transform.flip({
super.key,
bool flipX = false,
bool flipY = false,
this.origin,
this.transformHitTests = true,
this.filterQuality,
super.child,
}) : alignment = Alignment.center,
transform = Matrix4.diagonal3Values(flipX ? -1.0 : 1.0, flipY ? -1.0 : 1.0, 1.0);
// Computes a rotation matrix for an angle in radians, attempting to keep rotations
// at integral values for angles of 0, π/2, π, 3π/2.
static Matrix4 _computeRotation(double radians) {
assert(radians.isFinite, 'Cannot compute the rotation matrix for a non-finite angle: $radians');
if (radians == 0.0) {
return Matrix4.identity();
}
final double sin = math.sin(radians);
if (sin == 1.0) {
return _createZRotation(1.0, 0.0);
}
if (sin == -1.0) {
return _createZRotation(-1.0, 0.0);
}
final double cos = math.cos(radians);
if (cos == -1.0) {
return _createZRotation(0.0, -1.0);
}
return _createZRotation(sin, cos);
}
static Matrix4 _createZRotation(double sin, double cos) {
final Matrix4 result = Matrix4.zero();
result.storage[0] = cos;
result.storage[1] = sin;
result.storage[4] = -sin;
result.storage[5] = cos;
result.storage[10] = 1.0;
result.storage[15] = 1.0;
return result;
}
/// The matrix to transform the child by during painting.
final Matrix4 transform;
/// The origin of the coordinate system (relative to the upper left corner of
/// this render object) in which to apply the matrix.
///
/// Setting an origin is equivalent to conjugating the transform matrix by a
/// translation. This property is provided just for convenience.
final Offset? origin;
/// The alignment of the origin, relative to the size of the box.
///
/// This is equivalent to setting an origin based on the size of the box.
/// If it is specified at the same time as the [origin], both are applied.
///
/// An [AlignmentDirectional.centerStart] value is the same as an [Alignment]
/// whose [Alignment.x] value is `-1.0` if [Directionality.of] returns
/// [TextDirection.ltr], and `1.0` if [Directionality.of] returns
/// [TextDirection.rtl]. Similarly [AlignmentDirectional.centerEnd] is the
/// same as an [Alignment] whose [Alignment.x] value is `1.0` if
/// [Directionality.of] returns [TextDirection.ltr], and `-1.0` if
/// [Directionality.of] returns [TextDirection.rtl].
final AlignmentGeometry? alignment;
/// Whether to apply the transformation when performing hit tests.
final bool transformHitTests;
/// The filter quality with which to apply the transform as a bitmap operation.
///
/// {@template flutter.widgets.Transform.optional.FilterQuality}
/// The transform will be applied by re-rendering the child if [filterQuality] is null,
/// otherwise it controls the quality of an [ImageFilter.matrix] applied to a bitmap
/// rendering of the child.
/// {@endtemplate}
final FilterQuality? filterQuality;
@override
RenderTransform createRenderObject(BuildContext context) {
return RenderTransform(
transform: transform,
origin: origin,
alignment: alignment,
textDirection: Directionality.maybeOf(context),
transformHitTests: transformHitTests,
filterQuality: filterQuality,
);
}
@override
void updateRenderObject(BuildContext context, RenderTransform renderObject) {
renderObject
..transform = transform
..origin = origin
..alignment = alignment
..textDirection = Directionality.maybeOf(context)
..transformHitTests = transformHitTests
..filterQuality = filterQuality;
}
}
/// A widget that can be targeted by a [CompositedTransformFollower].
///
/// When this widget is composited during the compositing phase (which comes
/// after the paint phase, as described in [WidgetsBinding.drawFrame]), it
/// updates the [link] object so that any [CompositedTransformFollower] widgets
/// that are subsequently composited in the same frame and were given the same
/// [LayerLink] can position themselves at the same screen location.
///
/// A single [CompositedTransformTarget] can be followed by multiple
/// [CompositedTransformFollower] widgets.
///
/// The [CompositedTransformTarget] must come earlier in the paint order than
/// any linked [CompositedTransformFollower]s.
///
/// See also:
///
/// * [CompositedTransformFollower], the widget that can target this one.
/// * [LeaderLayer], the layer that implements this widget's logic.
class CompositedTransformTarget extends SingleChildRenderObjectWidget {
/// Creates a composited transform target widget.
///
/// The [link] property must not be currently used by any other
/// [CompositedTransformTarget] object that is in the tree.
const CompositedTransformTarget({
super.key,
required this.link,
super.child,
});
/// The link object that connects this [CompositedTransformTarget] with one or
/// more [CompositedTransformFollower]s.
///
/// The link must not be associated with another [CompositedTransformTarget]
/// that is also being painted.
final LayerLink link;
@override
RenderLeaderLayer createRenderObject(BuildContext context) {
return RenderLeaderLayer(
link: link,
);
}
@override
void updateRenderObject(BuildContext context, RenderLeaderLayer renderObject) {
renderObject.link = link;
}
}
/// A widget that follows a [CompositedTransformTarget].
///
/// When this widget is composited during the compositing phase (which comes
/// after the paint phase, as described in [WidgetsBinding.drawFrame]), it
/// applies a transformation that brings [targetAnchor] of the linked
/// [CompositedTransformTarget] and [followerAnchor] of this widget together.
/// The two anchor points will have the same global coordinates, unless [offset]
/// is not [Offset.zero], in which case [followerAnchor] will be offset by
/// [offset] in the linked [CompositedTransformTarget]'s coordinate space.
///
/// The [LayerLink] object used as the [link] must be the same object as that
/// provided to the matching [CompositedTransformTarget].
///
/// The [CompositedTransformTarget] must come earlier in the paint order than
/// this [CompositedTransformFollower].
///
/// Hit testing on descendants of this widget will only work if the target
/// position is within the box that this widget's parent considers to be
/// hittable. If the parent covers the screen, this is trivially achievable, so
/// this widget is usually used as the root of an [OverlayEntry] in an app-wide
/// [Overlay] (e.g. as created by the [MaterialApp] widget's [Navigator]).
///
/// See also:
///
/// * [CompositedTransformTarget], the widget that this widget can target.
/// * [FollowerLayer], the layer that implements this widget's logic.
/// * [Transform], which applies an arbitrary transform to a child.
class CompositedTransformFollower extends SingleChildRenderObjectWidget {
/// Creates a composited transform target widget.
///
/// If the [link] property was also provided to a [CompositedTransformTarget],
/// that widget must come earlier in the paint order.
///
/// The [showWhenUnlinked] and [offset] properties must also not be null.
const CompositedTransformFollower({
super.key,
required this.link,
this.showWhenUnlinked = true,
this.offset = Offset.zero,
this.targetAnchor = Alignment.topLeft,
this.followerAnchor = Alignment.topLeft,
super.child,
});
/// The link object that connects this [CompositedTransformFollower] with a
/// [CompositedTransformTarget].
final LayerLink link;
/// Whether to show the widget's contents when there is no corresponding
/// [CompositedTransformTarget] with the same [link].
///
/// When the widget is linked, the child is positioned such that it has the
/// same global position as the linked [CompositedTransformTarget].
///
/// When the widget is not linked, then: if [showWhenUnlinked] is true, the
/// child is visible and not repositioned; if it is false, then child is
/// hidden.
final bool showWhenUnlinked;
/// The anchor point on the linked [CompositedTransformTarget] that
/// [followerAnchor] will line up with.
///
/// {@template flutter.widgets.CompositedTransformFollower.targetAnchor}
/// For example, when [targetAnchor] and [followerAnchor] are both
/// [Alignment.topLeft], this widget will be top left aligned with the linked
/// [CompositedTransformTarget]. When [targetAnchor] is
/// [Alignment.bottomLeft] and [followerAnchor] is [Alignment.topLeft], this
/// widget will be left aligned with the linked [CompositedTransformTarget],
/// and its top edge will line up with the [CompositedTransformTarget]'s
/// bottom edge.
/// {@endtemplate}
///
/// Defaults to [Alignment.topLeft].
final Alignment targetAnchor;
/// The anchor point on this widget that will line up with [targetAnchor] on
/// the linked [CompositedTransformTarget].
///
/// {@macro flutter.widgets.CompositedTransformFollower.targetAnchor}
///
/// Defaults to [Alignment.topLeft].
final Alignment followerAnchor;
/// The additional offset to apply to the [targetAnchor] of the linked
/// [CompositedTransformTarget] to obtain this widget's [followerAnchor]
/// position.
final Offset offset;
@override
RenderFollowerLayer createRenderObject(BuildContext context) {
return RenderFollowerLayer(
link: link,
showWhenUnlinked: showWhenUnlinked,
offset: offset,
leaderAnchor: targetAnchor,
followerAnchor: followerAnchor,
);
}
@override
void updateRenderObject(BuildContext context, RenderFollowerLayer renderObject) {
renderObject
..link = link
..showWhenUnlinked = showWhenUnlinked
..offset = offset
..leaderAnchor = targetAnchor
..followerAnchor = followerAnchor;
}
}
/// Scales and positions its child within itself according to [fit].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=T4Uehk3_wlY}
///
/// {@tool dartpad}
/// In this example, the [Placeholder] is stretched to fill the entire
/// [Container]. Try changing the fit types to see the effect on the layout of
/// the [Placeholder].
///
/// ** See code in examples/api/lib/widgets/basic/fitted_box.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [Transform], which applies an arbitrary transform to its child widget at
/// paint time.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class FittedBox extends SingleChildRenderObjectWidget {
/// Creates a widget that scales and positions its child within itself according to [fit].
const FittedBox({
super.key,
this.fit = BoxFit.contain,
this.alignment = Alignment.center,
this.clipBehavior = Clip.none,
super.child,
});
/// How to inscribe the child into the space allocated during layout.
final BoxFit fit;
/// How to align the child within its parent's bounds.
///
/// An alignment of (-1.0, -1.0) aligns the child to the top-left corner of its
/// parent's bounds. An alignment of (1.0, 0.0) aligns the child to the middle
/// of the right edge of its parent's bounds.
///
/// Defaults to [Alignment.center].
///
/// See also:
///
/// * [Alignment], a class with convenient constants typically used to
/// specify an [AlignmentGeometry].
/// * [AlignmentDirectional], like [Alignment] for specifying alignments
/// relative to text direction.
final AlignmentGeometry alignment;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.none].
final Clip clipBehavior;
@override
RenderFittedBox createRenderObject(BuildContext context) {
return RenderFittedBox(
fit: fit,
alignment: alignment,
textDirection: Directionality.maybeOf(context),
clipBehavior: clipBehavior,
);
}
@override
void updateRenderObject(BuildContext context, RenderFittedBox renderObject) {
renderObject
..fit = fit
..alignment = alignment
..textDirection = Directionality.maybeOf(context)
..clipBehavior = clipBehavior;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<BoxFit>('fit', fit));
properties.add(DiagnosticsProperty<AlignmentGeometry>('alignment', alignment));
}
}
/// Applies a translation transformation before painting its child.
///
/// The translation is expressed as a [Offset] scaled to the child's size. For
/// example, an [Offset] with a `dx` of 0.25 will result in a horizontal
/// translation of one quarter the width of the child.
///
/// Hit tests will only be detected inside the bounds of the
/// [FractionalTranslation], even if the contents are offset such that
/// they overflow.
///
/// See also:
///
/// * [Transform], which applies an arbitrary transform to its child widget at
/// paint time.
/// * [Transform.translate], which applies an absolute offset translation
/// transformation instead of an offset scaled to the child.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class FractionalTranslation extends SingleChildRenderObjectWidget {
/// Creates a widget that translates its child's painting.
const FractionalTranslation({
super.key,
required this.translation,
this.transformHitTests = true,
super.child,
});
/// The translation to apply to the child, scaled to the child's size.
///
/// For example, an [Offset] with a `dx` of 0.25 will result in a horizontal
/// translation of one quarter the width of the child.
final Offset translation;
/// Whether to apply the translation when performing hit tests.
final bool transformHitTests;
@override
RenderFractionalTranslation createRenderObject(BuildContext context) {
return RenderFractionalTranslation(
translation: translation,
transformHitTests: transformHitTests,
);
}
@override
void updateRenderObject(BuildContext context, RenderFractionalTranslation renderObject) {
renderObject
..translation = translation
..transformHitTests = transformHitTests;
}
}
/// A widget that rotates its child by a integral number of quarter turns.
///
/// Unlike [Transform], which applies a transform just prior to painting,
/// this object applies its rotation prior to layout, which means the entire
/// rotated box consumes only as much space as required by the rotated child.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=BFE6_UglLfQ}
///
/// {@tool snippet}
///
/// This snippet rotates the child (some [Text]) so that it renders from bottom
/// to top, like an axis label on a graph:
///
/// ```dart
/// const RotatedBox(
/// quarterTurns: 3,
/// child: Text('Hello World!'),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [Transform], which is a paint effect that allows you to apply an
/// arbitrary transform to a child.
/// * [Transform.rotate], which applies a rotation paint effect.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class RotatedBox extends SingleChildRenderObjectWidget {
/// A widget that rotates its child.
const RotatedBox({
super.key,
required this.quarterTurns,
super.child,
});
/// The number of clockwise quarter turns the child should be rotated.
final int quarterTurns;
@override
RenderRotatedBox createRenderObject(BuildContext context) => RenderRotatedBox(quarterTurns: quarterTurns);
@override
void updateRenderObject(BuildContext context, RenderRotatedBox renderObject) {
renderObject.quarterTurns = quarterTurns;
}
}
/// A widget that insets its child by the given padding.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=oD5RtLhhubg}
///
/// When passing layout constraints to its child, padding shrinks the
/// constraints by the given padding, causing the child to layout at a smaller
/// size. Padding then sizes itself to its child's size, inflated by the
/// padding, effectively creating empty space around the child.
///
/// {@tool snippet}
///
/// This snippet creates "Hello World!" [Text] inside a [Card] that is indented
/// by sixteen pixels in each direction.
///
/// 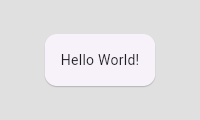
///
/// ```dart
/// const Card(
/// child: Padding(
/// padding: EdgeInsets.all(16.0),
/// child: Text('Hello World!'),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// ## Design discussion
///
/// ### Why use a [Padding] widget rather than a [Container] with a [Container.padding] property?
///
/// There isn't really any difference between the two. If you supply a
/// [Container.padding] argument, [Container] builds a [Padding] widget
/// for you.
///
/// [Container] doesn't implement its properties directly. Instead, [Container]
/// combines a number of simpler widgets together into a convenient package. For
/// example, the [Container.padding] property causes the container to build a
/// [Padding] widget and the [Container.decoration] property causes the
/// container to build a [DecoratedBox] widget. If you find [Container]
/// convenient, feel free to use it. If not, feel free to build these simpler
/// widgets in whatever combination meets your needs.
///
/// In fact, the majority of widgets in Flutter are combinations of other
/// simpler widgets. Composition, rather than inheritance, is the primary
/// mechanism for building up widgets.
///
/// See also:
///
/// * [EdgeInsets], the class that is used to describe the padding dimensions.
/// * [AnimatedPadding], which animates changes in [padding] over a given
/// duration.
/// * [SliverPadding], the sliver equivalent of this widget.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class Padding extends SingleChildRenderObjectWidget {
/// Creates a widget that insets its child.
const Padding({
super.key,
required this.padding,
super.child,
});
/// The amount of space by which to inset the child.
final EdgeInsetsGeometry padding;
@override
RenderPadding createRenderObject(BuildContext context) {
return RenderPadding(
padding: padding,
textDirection: Directionality.maybeOf(context),
);
}
@override
void updateRenderObject(BuildContext context, RenderPadding renderObject) {
renderObject
..padding = padding
..textDirection = Directionality.maybeOf(context);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('padding', padding));
}
}
/// A widget that aligns its child within itself and optionally sizes itself
/// based on the child's size.
///
/// For example, to align a box at the bottom right, you would pass this box a
/// tight constraint that is bigger than the child's natural size,
/// with an alignment of [Alignment.bottomRight].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=g2E7yl3MwMk}
///
/// This widget will be as big as possible if its dimensions are constrained and
/// [widthFactor] and [heightFactor] are null. If a dimension is unconstrained
/// and the corresponding size factor is null then the widget will match its
/// child's size in that dimension. If a size factor is non-null then the
/// corresponding dimension of this widget will be the product of the child's
/// dimension and the size factor. For example if widthFactor is 2.0 then
/// the width of this widget will always be twice its child's width.
///
/// {@tool snippet}
/// The [Align] widget in this example uses one of the defined constants from
/// [Alignment], [Alignment.topRight]. This places the [FlutterLogo] in the top
/// right corner of the parent blue [Container].
///
/// 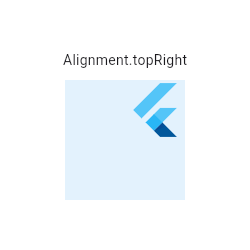
///
/// ```dart
/// Center(
/// child: Container(
/// height: 120.0,
/// width: 120.0,
/// color: Colors.blue[50],
/// child: const Align(
/// alignment: Alignment.topRight,
/// child: FlutterLogo(
/// size: 60,
/// ),
/// ),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// ## How it works
///
/// The [alignment] property describes a point in the `child`'s coordinate system
/// and a different point in the coordinate system of this widget. The [Align]
/// widget positions the `child` such that both points are lined up on top of
/// each other.
///
/// {@tool snippet}
/// The [Alignment] used in the following example defines two points:
///
/// * (0.2 * width of [FlutterLogo]/2 + width of [FlutterLogo]/2, 0.6 * height
/// of [FlutterLogo]/2 + height of [FlutterLogo]/2) = (36.0, 48.0) in the
/// coordinate system of the [FlutterLogo].
/// * (0.2 * width of [Align]/2 + width of [Align]/2, 0.6 * height
/// of [Align]/2 + height of [Align]/2) = (72.0, 96.0) in the
/// coordinate system of the [Align] widget (blue area).
///
/// The [Align] widget positions the [FlutterLogo] such that the two points are on
/// top of each other. In this example, the top left of the [FlutterLogo] will
/// be placed at (72.0, 96.0) - (36.0, 48.0) = (36.0, 48.0) from the top left of
/// the [Align] widget.
///
/// 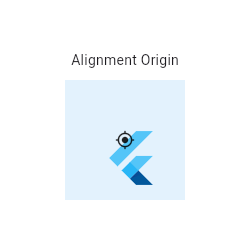
///
/// ```dart
/// Center(
/// child: Container(
/// height: 120.0,
/// width: 120.0,
/// color: Colors.blue[50],
/// child: const Align(
/// alignment: Alignment(0.2, 0.6),
/// child: FlutterLogo(
/// size: 60,
/// ),
/// ),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// The [FractionalOffset] used in the following example defines two points:
///
/// * (0.2 * width of [FlutterLogo], 0.6 * height of [FlutterLogo]) = (12.0, 36.0)
/// in the coordinate system of the [FlutterLogo].
/// * (0.2 * width of [Align], 0.6 * height of [Align]) = (24.0, 72.0) in the
/// coordinate system of the [Align] widget (blue area).
///
/// The [Align] widget positions the [FlutterLogo] such that the two points are on
/// top of each other. In this example, the top left of the [FlutterLogo] will
/// be placed at (24.0, 72.0) - (12.0, 36.0) = (12.0, 36.0) from the top left of
/// the [Align] widget.
///
/// The [FractionalOffset] class uses a coordinate system with an origin in the top-left
/// corner of the [Container] in difference to the center-oriented system used in
/// the example above with [Alignment].
///
/// 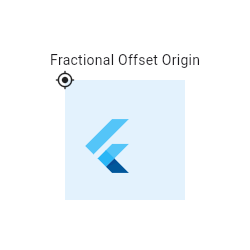
///
/// ```dart
/// Center(
/// child: Container(
/// height: 120.0,
/// width: 120.0,
/// color: Colors.blue[50],
/// child: const Align(
/// alignment: FractionalOffset(0.2, 0.6),
/// child: FlutterLogo(
/// size: 60,
/// ),
/// ),
/// ),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [AnimatedAlign], which animates changes in [alignment] smoothly over a
/// given duration.
/// * [CustomSingleChildLayout], which uses a delegate to control the layout of
/// a single child.
/// * [Center], which is the same as [Align] but with the [alignment] always
/// set to [Alignment.center].
/// * [FractionallySizedBox], which sizes its child based on a fraction of its
/// own size and positions the child according to an [Alignment] value.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class Align extends SingleChildRenderObjectWidget {
/// Creates an alignment widget.
///
/// The alignment defaults to [Alignment.center].
const Align({
super.key,
this.alignment = Alignment.center,
this.widthFactor,
this.heightFactor,
super.child,
}) : assert(widthFactor == null || widthFactor >= 0.0),
assert(heightFactor == null || heightFactor >= 0.0);
/// How to align the child.
///
/// The x and y values of the [Alignment] control the horizontal and vertical
/// alignment, respectively. An x value of -1.0 means that the left edge of
/// the child is aligned with the left edge of the parent whereas an x value
/// of 1.0 means that the right edge of the child is aligned with the right
/// edge of the parent. Other values interpolate (and extrapolate) linearly.
/// For example, a value of 0.0 means that the center of the child is aligned
/// with the center of the parent.
///
/// See also:
///
/// * [Alignment], which has more details and some convenience constants for
/// common positions.
/// * [AlignmentDirectional], which has a horizontal coordinate orientation
/// that depends on the [TextDirection].
final AlignmentGeometry alignment;
/// If non-null, sets its width to the child's width multiplied by this factor.
///
/// Can be both greater and less than 1.0 but must be non-negative.
final double? widthFactor;
/// If non-null, sets its height to the child's height multiplied by this factor.
///
/// Can be both greater and less than 1.0 but must be non-negative.
final double? heightFactor;
@override
RenderPositionedBox createRenderObject(BuildContext context) {
return RenderPositionedBox(
alignment: alignment,
widthFactor: widthFactor,
heightFactor: heightFactor,
textDirection: Directionality.maybeOf(context),
);
}
@override
void updateRenderObject(BuildContext context, RenderPositionedBox renderObject) {
renderObject
..alignment = alignment
..widthFactor = widthFactor
..heightFactor = heightFactor
..textDirection = Directionality.maybeOf(context);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<AlignmentGeometry>('alignment', alignment));
properties.add(DoubleProperty('widthFactor', widthFactor, defaultValue: null));
properties.add(DoubleProperty('heightFactor', heightFactor, defaultValue: null));
}
}
/// A widget that centers its child within itself.
///
/// This widget will be as big as possible if its dimensions are constrained and
/// [widthFactor] and [heightFactor] are null. If a dimension is unconstrained
/// and the corresponding size factor is null then the widget will match its
/// child's size in that dimension. If a size factor is non-null then the
/// corresponding dimension of this widget will be the product of the child's
/// dimension and the size factor. For example if widthFactor is 2.0 then
/// the width of this widget will always be twice its child's width.
///
/// See also:
///
/// * [Align], which lets you arbitrarily position a child within itself,
/// rather than just centering it.
/// * [Row], a widget that displays its children in a horizontal array.
/// * [Column], a widget that displays its children in a vertical array.
/// * [Container], a convenience widget that combines common painting,
/// positioning, and sizing widgets.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class Center extends Align {
/// Creates a widget that centers its child.
const Center({ super.key, super.widthFactor, super.heightFactor, super.child });
}
/// A widget that defers the layout of its single child to a delegate.
///
/// The delegate can determine the layout constraints for the child and can
/// decide where to position the child. The delegate can also determine the size
/// of the parent, but the size of the parent cannot depend on the size of the
/// child.
///
/// See also:
///
/// * [SingleChildLayoutDelegate], which controls the layout of the child.
/// * [Align], which sizes itself based on its child's size and positions
/// the child according to an [Alignment] value.
/// * [FractionallySizedBox], which sizes its child based on a fraction of its own
/// size and positions the child according to an [Alignment] value.
/// * [CustomMultiChildLayout], which uses a delegate to position multiple
/// children.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class CustomSingleChildLayout extends SingleChildRenderObjectWidget {
/// Creates a custom single child layout.
const CustomSingleChildLayout({
super.key,
required this.delegate,
super.child,
});
/// The delegate that controls the layout of the child.
final SingleChildLayoutDelegate delegate;
@override
RenderCustomSingleChildLayoutBox createRenderObject(BuildContext context) {
return RenderCustomSingleChildLayoutBox(delegate: delegate);
}
@override
void updateRenderObject(BuildContext context, RenderCustomSingleChildLayoutBox renderObject) {
renderObject.delegate = delegate;
}
}
/// Metadata for identifying children in a [CustomMultiChildLayout].
///
/// The [MultiChildLayoutDelegate.hasChild],
/// [MultiChildLayoutDelegate.layoutChild], and
/// [MultiChildLayoutDelegate.positionChild] methods use these identifiers.
class LayoutId extends ParentDataWidget<MultiChildLayoutParentData> {
/// Marks a child with a layout identifier.
LayoutId({
Key? key,
required this.id,
required super.child,
}) : super(key: key ?? ValueKey<Object>(id));
/// An object representing the identity of this child.
///
/// The [id] needs to be unique among the children that the
/// [CustomMultiChildLayout] manages.
final Object id;
@override
void applyParentData(RenderObject renderObject) {
assert(renderObject.parentData is MultiChildLayoutParentData);
final MultiChildLayoutParentData parentData = renderObject.parentData! as MultiChildLayoutParentData;
if (parentData.id != id) {
parentData.id = id;
final RenderObject? targetParent = renderObject.parent;
if (targetParent is RenderObject) {
targetParent.markNeedsLayout();
}
}
}
@override
Type get debugTypicalAncestorWidgetClass => CustomMultiChildLayout;
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<Object>('id', id));
}
}
/// A widget that uses a delegate to size and position multiple children.
///
/// The delegate can determine the layout constraints for each child and can
/// decide where to position each child. The delegate can also determine the
/// size of the parent, but the size of the parent cannot depend on the sizes of
/// the children.
///
/// [CustomMultiChildLayout] is appropriate when there are complex relationships
/// between the size and positioning of multiple widgets. To control the
/// layout of a single child, [CustomSingleChildLayout] is more appropriate. For
/// simple cases, such as aligning a widget to one or another edge, the [Stack]
/// widget is more appropriate.
///
/// Each child must be wrapped in a [LayoutId] widget to identify the widget for
/// the delegate.
///
/// {@tool dartpad}
/// This example shows a [CustomMultiChildLayout] widget being used to lay out
/// colored blocks from start to finish in a cascade that has some overlap.
///
/// It responds to changes in [Directionality] by re-laying out its children.
///
/// ** See code in examples/api/lib/widgets/basic/custom_multi_child_layout.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [MultiChildLayoutDelegate], for details about how to control the layout of
/// the children.
/// * [CustomSingleChildLayout], which uses a delegate to control the layout of
/// a single child.
/// * [Stack], which arranges children relative to the edges of the container.
/// * [Flow], which provides paint-time control of its children using transform
/// matrices.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class CustomMultiChildLayout extends MultiChildRenderObjectWidget {
/// Creates a custom multi-child layout.
const CustomMultiChildLayout({
super.key,
required this.delegate,
super.children,
});
/// The delegate that controls the layout of the children.
final MultiChildLayoutDelegate delegate;
@override
RenderCustomMultiChildLayoutBox createRenderObject(BuildContext context) {
return RenderCustomMultiChildLayoutBox(delegate: delegate);
}
@override
void updateRenderObject(BuildContext context, RenderCustomMultiChildLayoutBox renderObject) {
renderObject.delegate = delegate;
}
}
/// A box with a specified size.
///
/// If given a child, this widget forces it to have a specific width and/or height.
/// These values will be ignored if this widget's parent does not permit them.
/// For example, this happens if the parent is the screen (forces the child to
/// be the same size as the parent), or another [SizedBox] (forces its child to
/// have a specific width and/or height). This can be remedied by wrapping the
/// child [SizedBox] in a widget that does permit it to be any size up to the
/// size of the parent, such as [Center] or [Align].
///
/// If either the width or height is null, this widget will try to size itself to
/// match the child's size in that dimension. If the child's size depends on the
/// size of its parent, the height and width must be provided.
///
/// If not given a child, [SizedBox] will try to size itself as close to the
/// specified height and width as possible given the parent's constraints. If
/// [height] or [width] is null or unspecified, it will be treated as zero.
///
/// The [SizedBox.expand] constructor can be used to make a [SizedBox] that
/// sizes itself to fit the parent. It is equivalent to setting [width] and
/// [height] to [double.infinity].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=EHPu_DzRfqA}
///
/// {@tool snippet}
///
/// This snippet makes the child widget (a [Card] with some [Text]) have the
/// exact size 200x300, parental constraints permitting:
///
/// ```dart
/// const SizedBox(
/// width: 200.0,
/// height: 300.0,
/// child: Card(child: Text('Hello World!')),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [ConstrainedBox], a more generic version of this class that takes
/// arbitrary [BoxConstraints] instead of an explicit width and height.
/// * [UnconstrainedBox], a container that tries to let its child draw without
/// constraints.
/// * [FractionallySizedBox], a widget that sizes its child to a fraction of
/// the total available space.
/// * [AspectRatio], a widget that attempts to fit within the parent's
/// constraints while also sizing its child to match a given aspect ratio.
/// * [FittedBox], which sizes and positions its child widget to fit the parent
/// according to a given [BoxFit] discipline.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
/// * [Understanding constraints](https://flutter.dev/docs/development/ui/layout/constraints),
/// an in-depth article about layout in Flutter.
class SizedBox extends SingleChildRenderObjectWidget {
/// Creates a fixed size box. The [width] and [height] parameters can be null
/// to indicate that the size of the box should not be constrained in
/// the corresponding dimension.
const SizedBox({ super.key, this.width, this.height, super.child });
/// Creates a box that will become as large as its parent allows.
const SizedBox.expand({ super.key, super.child })
: width = double.infinity,
height = double.infinity;
/// Creates a box that will become as small as its parent allows.
const SizedBox.shrink({ super.key, super.child })
: width = 0.0,
height = 0.0;
/// Creates a box with the specified size.
SizedBox.fromSize({ super.key, super.child, Size? size })
: width = size?.width,
height = size?.height;
/// Creates a box whose [width] and [height] are equal.
const SizedBox.square({super.key, super.child, double? dimension})
: width = dimension,
height = dimension;
/// If non-null, requires the child to have exactly this width.
final double? width;
/// If non-null, requires the child to have exactly this height.
final double? height;
@override
RenderConstrainedBox createRenderObject(BuildContext context) {
return RenderConstrainedBox(
additionalConstraints: _additionalConstraints,
);
}
BoxConstraints get _additionalConstraints {
return BoxConstraints.tightFor(width: width, height: height);
}
@override
void updateRenderObject(BuildContext context, RenderConstrainedBox renderObject) {
renderObject.additionalConstraints = _additionalConstraints;
}
@override
String toStringShort() {
final String type;
if (width == double.infinity && height == double.infinity) {
type = '${objectRuntimeType(this, 'SizedBox')}.expand';
} else if (width == 0.0 && height == 0.0) {
type = '${objectRuntimeType(this, 'SizedBox')}.shrink';
} else {
type = objectRuntimeType(this, 'SizedBox');
}
return key == null ? type : '$type-$key';
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
final DiagnosticLevel level;
if ((width == double.infinity && height == double.infinity) ||
(width == 0.0 && height == 0.0)) {
level = DiagnosticLevel.hidden;
} else {
level = DiagnosticLevel.info;
}
properties.add(DoubleProperty('width', width, defaultValue: null, level: level));
properties.add(DoubleProperty('height', height, defaultValue: null, level: level));
}
}
/// A widget that imposes additional constraints on its child.
///
/// For example, if you wanted [child] to have a minimum height of 50.0 logical
/// pixels, you could use `const BoxConstraints(minHeight: 50.0)` as the
/// [constraints].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=o2KveVr7adg}
///
/// {@tool snippet}
///
/// This snippet makes the child widget (a [Card] with some [Text]) fill the
/// parent, by applying [BoxConstraints.expand] constraints:
///
/// ```dart
/// ConstrainedBox(
/// constraints: const BoxConstraints.expand(),
/// child: const Card(child: Text('Hello World!')),
/// )
/// ```
/// {@end-tool}
///
/// The same behavior can be obtained using the [SizedBox.expand] widget.
///
/// See also:
///
/// * [BoxConstraints], the class that describes constraints.
/// * [UnconstrainedBox], a container that tries to let its child draw without
/// constraints.
/// * [SizedBox], which lets you specify tight constraints by explicitly
/// specifying the height or width.
/// * [FractionallySizedBox], which sizes its child based on a fraction of its
/// own size and positions the child according to an [Alignment] value.
/// * [AspectRatio], a widget that attempts to fit within the parent's
/// constraints while also sizing its child to match a given aspect ratio.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class ConstrainedBox extends SingleChildRenderObjectWidget {
/// Creates a widget that imposes additional constraints on its child.
ConstrainedBox({
super.key,
required this.constraints,
super.child,
}) : assert(constraints.debugAssertIsValid());
/// The additional constraints to impose on the child.
final BoxConstraints constraints;
@override
RenderConstrainedBox createRenderObject(BuildContext context) {
return RenderConstrainedBox(additionalConstraints: constraints);
}
@override
void updateRenderObject(BuildContext context, RenderConstrainedBox renderObject) {
renderObject.additionalConstraints = constraints;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<BoxConstraints>('constraints', constraints, showName: false));
}
}
/// A container widget that applies an arbitrary transform to its constraints,
/// and sizes its child using the resulting [BoxConstraints], optionally
/// clipping, or treating the overflow as an error.
///
/// This container sizes its child using a [BoxConstraints] created by applying
/// [constraintsTransform] to its own constraints. This container will then
/// attempt to adopt the same size, within the limits of its own constraints. If
/// it ends up with a different size, it will align the child based on
/// [alignment]. If the container cannot expand enough to accommodate the entire
/// child, the child will be clipped if [clipBehavior] is not [Clip.none].
///
/// In debug mode, if [clipBehavior] is [Clip.none] and the child overflows the
/// container, a warning will be printed on the console, and black and yellow
/// striped areas will appear where the overflow occurs.
///
/// When [child] is null, this widget becomes as small as possible and never
/// overflows.
///
/// This widget can be used to ensure some of [child]'s natural dimensions are
/// honored, and get an early warning otherwise during development. For
/// instance, if [child] requires a minimum height to fully display its content,
/// [constraintsTransform] can be set to [maxHeightUnconstrained], so that if
/// the parent [RenderObject] fails to provide enough vertical space, a warning
/// will be displayed in debug mode, while still allowing [child] to grow
/// vertically:
///
/// {@tool snippet}
/// In the following snippet, the [Card] is guaranteed to be at least as tall as
/// its "natural" height. Unlike [UnconstrainedBox], it will become taller if
/// its "natural" height is smaller than 40 px. If the [Container] isn't high
/// enough to show the full content of the [Card], in debug mode a warning will
/// be given.
///
/// ```dart
/// Container(
/// constraints: const BoxConstraints(minHeight: 40, maxHeight: 100),
/// alignment: Alignment.center,
/// child: const ConstraintsTransformBox(
/// constraintsTransform: ConstraintsTransformBox.maxHeightUnconstrained,
/// child: Card(child: Text('Hello World!')),
/// )
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [ConstrainedBox], which renders a box which imposes constraints
/// on its child.
/// * [OverflowBox], a widget that imposes additional constraints on its child,
/// and allows the child to overflow itself.
/// * [UnconstrainedBox] which allows its children to render themselves
/// unconstrained and expands to fit them.
class ConstraintsTransformBox extends SingleChildRenderObjectWidget {
/// Creates a widget that uses a function to transform the constraints it
/// passes to its child. If the child overflows the parent's constraints, a
/// warning will be given in debug mode.
///
/// The `debugTransformType` argument adds a debug label to this widget.
///
/// The `alignment`, `clipBehavior` and `constraintsTransform` arguments must
/// not be null.
const ConstraintsTransformBox({
super.key,
super.child,
this.textDirection,
this.alignment = Alignment.center,
required this.constraintsTransform,
this.clipBehavior = Clip.none,
String debugTransformType = '',
}) : _debugTransformLabel = debugTransformType;
/// A [BoxConstraintsTransform] that always returns its argument as-is (i.e.,
/// it is an identity function).
///
/// The [ConstraintsTransformBox] becomes a proxy widget that has no effect on
/// layout if [constraintsTransform] is set to this.
static BoxConstraints unmodified(BoxConstraints constraints) => constraints;
/// A [BoxConstraintsTransform] that always returns a [BoxConstraints] that
/// imposes no constraints on either dimension (i.e. `const BoxConstraints()`).
///
/// Setting [constraintsTransform] to this allows [child] to render at its
/// "natural" size (equivalent to an [UnconstrainedBox] with `constrainedAxis`
/// set to null).
static BoxConstraints unconstrained(BoxConstraints constraints) => const BoxConstraints();
/// A [BoxConstraintsTransform] that removes the width constraints from the
/// input.
///
/// Setting [constraintsTransform] to this allows [child] to render at its
/// "natural" width (equivalent to an [UnconstrainedBox] with
/// `constrainedAxis` set to [Axis.horizontal]).
static BoxConstraints widthUnconstrained(BoxConstraints constraints) => constraints.heightConstraints();
/// A [BoxConstraintsTransform] that removes the height constraints from the
/// input.
///
/// Setting [constraintsTransform] to this allows [child] to render at its
/// "natural" height (equivalent to an [UnconstrainedBox] with
/// `constrainedAxis` set to [Axis.vertical]).
static BoxConstraints heightUnconstrained(BoxConstraints constraints) => constraints.widthConstraints();
/// A [BoxConstraintsTransform] that removes the `maxHeight` constraint from
/// the input.
///
/// Setting [constraintsTransform] to this allows [child] to render at its
/// "natural" height or the `minHeight` of the incoming [BoxConstraints],
/// whichever is larger.
static BoxConstraints maxHeightUnconstrained(BoxConstraints constraints) => constraints.copyWith(maxHeight: double.infinity);
/// A [BoxConstraintsTransform] that removes the `maxWidth` constraint from
/// the input.
///
/// Setting [constraintsTransform] to this allows [child] to render at its
/// "natural" width or the `minWidth` of the incoming [BoxConstraints],
/// whichever is larger.
static BoxConstraints maxWidthUnconstrained(BoxConstraints constraints) => constraints.copyWith(maxWidth: double.infinity);
/// A [BoxConstraintsTransform] that removes both the `maxWidth` and the
/// `maxHeight` constraints from the input.
///
/// Setting [constraintsTransform] to this allows [child] to render at least
/// its "natural" size, and grow along an axis if the incoming
/// [BoxConstraints] has a larger minimum constraint on that axis.
static BoxConstraints maxUnconstrained(BoxConstraints constraints) => constraints.copyWith(maxWidth: double.infinity, maxHeight: double.infinity);
static final Map<BoxConstraintsTransform, String> _debugKnownTransforms = <BoxConstraintsTransform, String>{
unmodified: 'unmodified',
unconstrained: 'unconstrained',
widthUnconstrained: 'width constraints removed',
heightUnconstrained: 'height constraints removed',
maxWidthUnconstrained: 'maxWidth constraint removed',
maxHeightUnconstrained: 'maxHeight constraint removed',
maxUnconstrained: 'maxWidth & maxHeight constraints removed',
};
/// The text direction to use when interpreting the [alignment] if it is an
/// [AlignmentDirectional].
///
/// Defaults to null, in which case [Directionality.maybeOf] is used to determine
/// the text direction.
final TextDirection? textDirection;
/// The alignment to use when laying out the child, if it has a different size
/// than this widget.
///
/// If this is an [AlignmentDirectional], then [textDirection] must not be
/// null.
///
/// See also:
///
/// * [Alignment] for non-[Directionality]-aware alignments.
/// * [AlignmentDirectional] for [Directionality]-aware alignments.
final AlignmentGeometry alignment;
/// {@template flutter.widgets.constraintsTransform}
/// The function used to transform the incoming [BoxConstraints], to size
/// [child].
///
/// The function must return a [BoxConstraints] that is
/// [BoxConstraints.isNormalized].
///
/// See [ConstraintsTransformBox] for predefined common
/// [BoxConstraintsTransform]s.
/// {@endtemplate}
final BoxConstraintsTransform constraintsTransform;
/// {@macro flutter.material.Material.clipBehavior}
///
/// {@template flutter.widgets.ConstraintsTransformBox.clipBehavior}
/// In debug mode, if [clipBehavior] is [Clip.none], and the child overflows
/// its constraints, a warning will be printed on the console, and black and
/// yellow striped areas will appear where the overflow occurs. For other
/// values of [clipBehavior], the contents are clipped accordingly.
/// {@endtemplate}
///
/// Defaults to [Clip.none].
final Clip clipBehavior;
final String _debugTransformLabel;
@override
RenderConstraintsTransformBox createRenderObject(BuildContext context) {
return RenderConstraintsTransformBox(
textDirection: textDirection ?? Directionality.maybeOf(context),
alignment: alignment,
constraintsTransform: constraintsTransform,
clipBehavior: clipBehavior,
);
}
@override
void updateRenderObject(BuildContext context, covariant RenderConstraintsTransformBox renderObject) {
renderObject
..textDirection = textDirection ?? Directionality.maybeOf(context)
..constraintsTransform = constraintsTransform
..alignment = alignment
..clipBehavior = clipBehavior;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<AlignmentGeometry>('alignment', alignment));
properties.add(EnumProperty<TextDirection>('textDirection', textDirection, defaultValue: null));
final String? debugTransformLabel = _debugTransformLabel.isNotEmpty
? _debugTransformLabel
: _debugKnownTransforms[constraintsTransform];
if (debugTransformLabel != null) {
properties.add(DiagnosticsProperty<String>('constraints transform', debugTransformLabel));
}
}
}
/// A widget that imposes no constraints on its child, allowing it to render
/// at its "natural" size.
///
/// This allows a child to render at the size it would render if it were alone
/// on an infinite canvas with no constraints. This container will then attempt
/// to adopt the same size, within the limits of its own constraints. If it ends
/// up with a different size, it will align the child based on [alignment].
/// If the box cannot expand enough to accommodate the entire child, the
/// child will be clipped.
///
/// In debug mode, if the child overflows the container, a warning will be
/// printed on the console, and black and yellow striped areas will appear where
/// the overflow occurs.
///
/// See also:
///
/// * [ConstrainedBox], for a box which imposes constraints on its child.
/// * [Align], which loosens the constraints given to the child rather than
/// removing them entirely.
/// * [Container], a convenience widget that combines common painting,
/// positioning, and sizing widgets.
/// * [OverflowBox], a widget that imposes different constraints on its child
/// than it gets from its parent, possibly allowing the child to overflow
/// the parent.
/// * [ConstraintsTransformBox], a widget that sizes its child using a
/// transformed [BoxConstraints], and shows a warning if the child overflows
/// in debug mode.
class UnconstrainedBox extends StatelessWidget {
/// Creates a widget that imposes no constraints on its child, allowing it to
/// render at its "natural" size. If the child overflows the parents
/// constraints, a warning will be given in debug mode.
const UnconstrainedBox({
super.key,
this.child,
this.textDirection,
this.alignment = Alignment.center,
this.constrainedAxis,
this.clipBehavior = Clip.none,
});
/// The text direction to use when interpreting the [alignment] if it is an
/// [AlignmentDirectional].
final TextDirection? textDirection;
/// The alignment to use when laying out the child.
///
/// If this is an [AlignmentDirectional], then [textDirection] must not be
/// null.
///
/// See also:
///
/// * [Alignment] for non-[Directionality]-aware alignments.
/// * [AlignmentDirectional] for [Directionality]-aware alignments.
final AlignmentGeometry alignment;
/// The axis to retain constraints on, if any.
///
/// If not set, or set to null (the default), neither axis will retain its
/// constraints. If set to [Axis.vertical], then vertical constraints will
/// be retained, and if set to [Axis.horizontal], then horizontal constraints
/// will be retained.
final Axis? constrainedAxis;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.none].
final Clip clipBehavior;
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget? child;
BoxConstraintsTransform _axisToTransform(Axis? constrainedAxis) {
return switch (constrainedAxis) {
Axis.horizontal => ConstraintsTransformBox.heightUnconstrained,
Axis.vertical => ConstraintsTransformBox.widthUnconstrained,
null => ConstraintsTransformBox.unconstrained,
};
}
@override
Widget build(BuildContext context) {
return ConstraintsTransformBox(
textDirection: textDirection,
alignment: alignment,
clipBehavior: clipBehavior,
constraintsTransform: _axisToTransform(constrainedAxis),
child: child,
);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<AlignmentGeometry>('alignment', alignment));
properties.add(EnumProperty<Axis>('constrainedAxis', constrainedAxis, defaultValue: null));
properties.add(EnumProperty<TextDirection>('textDirection', textDirection, defaultValue: null));
}
}
/// A widget that sizes its child to a fraction of the total available space.
/// For more details about the layout algorithm, see
/// [RenderFractionallySizedOverflowBox].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=PEsY654EGZ0}
///
/// {@tool dartpad}
/// This sample shows a [FractionallySizedBox] whose one child is 50% of
/// the box's size per the width and height factor parameters, and centered
/// within that box by the alignment parameter.
///
/// ** See code in examples/api/lib/widgets/basic/fractionally_sized_box.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [Align], which sizes itself based on its child's size and positions
/// the child according to an [Alignment] value.
/// * [OverflowBox], a widget that imposes different constraints on its child
/// than it gets from its parent, possibly allowing the child to overflow the
/// parent.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class FractionallySizedBox extends SingleChildRenderObjectWidget {
/// Creates a widget that sizes its child to a fraction of the total available space.
///
/// If non-null, the [widthFactor] and [heightFactor] arguments must be
/// non-negative.
const FractionallySizedBox({
super.key,
this.alignment = Alignment.center,
this.widthFactor,
this.heightFactor,
super.child,
}) : assert(widthFactor == null || widthFactor >= 0.0),
assert(heightFactor == null || heightFactor >= 0.0);
/// {@template flutter.widgets.basic.fractionallySizedBox.widthFactor}
/// If non-null, the fraction of the incoming width given to the child.
///
/// If non-null, the child is given a tight width constraint that is the max
/// incoming width constraint multiplied by this factor.
///
/// If null, the incoming width constraints are passed to the child
/// unmodified.
/// {@endtemplate}
final double? widthFactor;
/// {@template flutter.widgets.basic.fractionallySizedBox.heightFactor}
/// If non-null, the fraction of the incoming height given to the child.
///
/// If non-null, the child is given a tight height constraint that is the max
/// incoming height constraint multiplied by this factor.
///
/// If null, the incoming height constraints are passed to the child
/// unmodified.
/// {@endtemplate}
final double? heightFactor;
/// {@template flutter.widgets.basic.fractionallySizedBox.alignment}
/// How to align the child.
///
/// The x and y values of the alignment control the horizontal and vertical
/// alignment, respectively. An x value of -1.0 means that the left edge of
/// the child is aligned with the left edge of the parent whereas an x value
/// of 1.0 means that the right edge of the child is aligned with the right
/// edge of the parent. Other values interpolate (and extrapolate) linearly.
/// For example, a value of 0.0 means that the center of the child is aligned
/// with the center of the parent.
///
/// Defaults to [Alignment.center].
///
/// See also:
///
/// * [Alignment], a class with convenient constants typically used to
/// specify an [AlignmentGeometry].
/// * [AlignmentDirectional], like [Alignment] for specifying alignments
/// relative to text direction.
/// {@endtemplate}
final AlignmentGeometry alignment;
@override
RenderFractionallySizedOverflowBox createRenderObject(BuildContext context) {
return RenderFractionallySizedOverflowBox(
alignment: alignment,
widthFactor: widthFactor,
heightFactor: heightFactor,
textDirection: Directionality.maybeOf(context),
);
}
@override
void updateRenderObject(BuildContext context, RenderFractionallySizedOverflowBox renderObject) {
renderObject
..alignment = alignment
..widthFactor = widthFactor
..heightFactor = heightFactor
..textDirection = Directionality.maybeOf(context);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<AlignmentGeometry>('alignment', alignment));
properties.add(DoubleProperty('widthFactor', widthFactor, defaultValue: null));
properties.add(DoubleProperty('heightFactor', heightFactor, defaultValue: null));
}
}
/// A box that limits its size only when it's unconstrained.
///
/// If this widget's maximum width is unconstrained then its child's width is
/// limited to [maxWidth]. Similarly, if this widget's maximum height is
/// unconstrained then its child's height is limited to [maxHeight].
///
/// This has the effect of giving the child a natural dimension in unbounded
/// environments. For example, by providing a [maxHeight] to a widget that
/// normally tries to be as big as possible, the widget will normally size
/// itself to fit its parent, but when placed in a vertical list, it will take
/// on the given height.
///
/// This is useful when composing widgets that normally try to match their
/// parents' size, so that they behave reasonably in lists (which are
/// unbounded).
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=uVki2CIzBTs}
///
/// See also:
///
/// * [ConstrainedBox], which applies its constraints in all cases, not just
/// when the incoming constraints are unbounded.
/// * [SizedBox], which lets you specify tight constraints by explicitly
/// specifying the height or width.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class LimitedBox extends SingleChildRenderObjectWidget {
/// Creates a box that limits its size only when it's unconstrained.
///
/// The [maxWidth] and [maxHeight] arguments must not be negative.
const LimitedBox({
super.key,
this.maxWidth = double.infinity,
this.maxHeight = double.infinity,
super.child,
}) : assert(maxWidth >= 0.0),
assert(maxHeight >= 0.0);
/// The maximum width limit to apply in the absence of a
/// [BoxConstraints.maxWidth] constraint.
final double maxWidth;
/// The maximum height limit to apply in the absence of a
/// [BoxConstraints.maxHeight] constraint.
final double maxHeight;
@override
RenderLimitedBox createRenderObject(BuildContext context) {
return RenderLimitedBox(
maxWidth: maxWidth,
maxHeight: maxHeight,
);
}
@override
void updateRenderObject(BuildContext context, RenderLimitedBox renderObject) {
renderObject
..maxWidth = maxWidth
..maxHeight = maxHeight;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('maxWidth', maxWidth, defaultValue: double.infinity));
properties.add(DoubleProperty('maxHeight', maxHeight, defaultValue: double.infinity));
}
}
/// A widget that imposes different constraints on its child than it gets
/// from its parent, possibly allowing the child to overflow the parent.
///
/// {@tool dartpad}
/// This example shows how an [OverflowBox] is used, and what its effect is.
///
/// ** See code in examples/api/lib/widgets/basic/overflowbox.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [RenderConstrainedOverflowBox] for details about how [OverflowBox] is
/// rendered.
/// * [SizedOverflowBox], a widget that is a specific size but passes its
/// original constraints through to its child, which may then overflow.
/// * [ConstrainedBox], a widget that imposes additional constraints on its
/// child.
/// * [UnconstrainedBox], a container that tries to let its child draw without
/// constraints.
/// * [SizedBox], a box with a specified size.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class OverflowBox extends SingleChildRenderObjectWidget {
/// Creates a widget that lets its child overflow itself.
const OverflowBox({
super.key,
this.alignment = Alignment.center,
this.minWidth,
this.maxWidth,
this.minHeight,
this.maxHeight,
this.fit = OverflowBoxFit.max,
super.child,
});
/// How to align the child.
///
/// The x and y values of the alignment control the horizontal and vertical
/// alignment, respectively. An x value of -1.0 means that the left edge of
/// the child is aligned with the left edge of the parent whereas an x value
/// of 1.0 means that the right edge of the child is aligned with the right
/// edge of the parent. Other values interpolate (and extrapolate) linearly.
/// For example, a value of 0.0 means that the center of the child is aligned
/// with the center of the parent.
///
/// Defaults to [Alignment.center].
///
/// See also:
///
/// * [Alignment], a class with convenient constants typically used to
/// specify an [AlignmentGeometry].
/// * [AlignmentDirectional], like [Alignment] for specifying alignments
/// relative to text direction.
final AlignmentGeometry alignment;
/// The minimum width constraint to give the child. Set this to null (the
/// default) to use the constraint from the parent instead.
final double? minWidth;
/// The maximum width constraint to give the child. Set this to null (the
/// default) to use the constraint from the parent instead.
final double? maxWidth;
/// The minimum height constraint to give the child. Set this to null (the
/// default) to use the constraint from the parent instead.
final double? minHeight;
/// The maximum height constraint to give the child. Set this to null (the
/// default) to use the constraint from the parent instead.
final double? maxHeight;
/// The way to size the render object.
///
/// This only affects scenario when the child does not indeed overflow.
/// If set to [OverflowBoxFit.deferToChild], the render object will size itself to
/// match the size of its child within the constraints of its parent or be
/// as small as the parent allows if no child is set. If set to
/// [OverflowBoxFit.max] (the default), the render object will size itself
/// to be as large as the parent allows.
final OverflowBoxFit fit;
@override
RenderConstrainedOverflowBox createRenderObject(BuildContext context) {
return RenderConstrainedOverflowBox(
alignment: alignment,
minWidth: minWidth,
maxWidth: maxWidth,
minHeight: minHeight,
maxHeight: maxHeight,
fit: fit,
textDirection: Directionality.maybeOf(context),
);
}
@override
void updateRenderObject(BuildContext context, RenderConstrainedOverflowBox renderObject) {
renderObject
..alignment = alignment
..minWidth = minWidth
..maxWidth = maxWidth
..minHeight = minHeight
..maxHeight = maxHeight
..fit = fit
..textDirection = Directionality.maybeOf(context);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<AlignmentGeometry>('alignment', alignment));
properties.add(DoubleProperty('minWidth', minWidth, defaultValue: null));
properties.add(DoubleProperty('maxWidth', maxWidth, defaultValue: null));
properties.add(DoubleProperty('minHeight', minHeight, defaultValue: null));
properties.add(DoubleProperty('maxHeight', maxHeight, defaultValue: null));
properties.add(EnumProperty<OverflowBoxFit>('fit', fit));
}
}
/// A widget that is a specific size but passes its original constraints
/// through to its child, which may then overflow.
///
/// See also:
///
/// * [OverflowBox], A widget that imposes different constraints on its child
/// than it gets from its parent, possibly allowing the child to overflow the
/// parent.
/// * [ConstrainedBox], a widget that imposes additional constraints on its
/// child.
/// * [UnconstrainedBox], a container that tries to let its child draw without
/// constraints.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class SizedOverflowBox extends SingleChildRenderObjectWidget {
/// Creates a widget of a given size that lets its child overflow.
const SizedOverflowBox({
super.key,
required this.size,
this.alignment = Alignment.center,
super.child,
});
/// How to align the child.
///
/// The x and y values of the alignment control the horizontal and vertical
/// alignment, respectively. An x value of -1.0 means that the left edge of
/// the child is aligned with the left edge of the parent whereas an x value
/// of 1.0 means that the right edge of the child is aligned with the right
/// edge of the parent. Other values interpolate (and extrapolate) linearly.
/// For example, a value of 0.0 means that the center of the child is aligned
/// with the center of the parent.
///
/// Defaults to [Alignment.center].
///
/// See also:
///
/// * [Alignment], a class with convenient constants typically used to
/// specify an [AlignmentGeometry].
/// * [AlignmentDirectional], like [Alignment] for specifying alignments
/// relative to text direction.
final AlignmentGeometry alignment;
/// The size this widget should attempt to be.
final Size size;
@override
RenderSizedOverflowBox createRenderObject(BuildContext context) {
return RenderSizedOverflowBox(
alignment: alignment,
requestedSize: size,
textDirection: Directionality.of(context),
);
}
@override
void updateRenderObject(BuildContext context, RenderSizedOverflowBox renderObject) {
renderObject
..alignment = alignment
..requestedSize = size
..textDirection = Directionality.of(context);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<AlignmentGeometry>('alignment', alignment));
properties.add(DiagnosticsProperty<Size>('size', size, defaultValue: null));
}
}
/// A widget that lays the child out as if it was in the tree, but without
/// painting anything, without making the child available for hit testing, and
/// without taking any room in the parent.
///
/// Offstage children are still active: they can receive focus and have keyboard
/// input directed to them.
///
/// Animations continue to run in offstage children, and therefore use battery
/// and CPU time, regardless of whether the animations end up being visible.
///
/// [Offstage] can be used to measure the dimensions of a widget without
/// bringing it on screen (yet). To hide a widget from view while it is not
/// needed, prefer removing the widget from the tree entirely rather than
/// keeping it alive in an [Offstage] subtree.
///
/// {@tool dartpad}
/// This example shows a [FlutterLogo] widget when the `_offstage` member field
/// is false, and hides it without any room in the parent when it is true. When
/// offstage, this app displays a button to get the logo size, which will be
/// displayed in a [SnackBar].
///
/// ** See code in examples/api/lib/widgets/basic/offstage.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [Visibility], which can hide a child more efficiently (albeit less
/// subtly).
/// * [TickerMode], which can be used to disable animations in a subtree.
/// * [SliverOffstage], the sliver version of this widget.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class Offstage extends SingleChildRenderObjectWidget {
/// Creates a widget that visually hides its child.
const Offstage({ super.key, this.offstage = true, super.child });
/// Whether the child is hidden from the rest of the tree.
///
/// If true, the child is laid out as if it was in the tree, but without
/// painting anything, without making the child available for hit testing, and
/// without taking any room in the parent.
///
/// Offstage children are still active: they can receive focus and have keyboard
/// input directed to them.
///
/// Animations continue to run in offstage children, and therefore use battery
/// and CPU time, regardless of whether the animations end up being visible.
///
/// If false, the child is included in the tree as normal.
final bool offstage;
@override
RenderOffstage createRenderObject(BuildContext context) => RenderOffstage(offstage: offstage);
@override
void updateRenderObject(BuildContext context, RenderOffstage renderObject) {
renderObject.offstage = offstage;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<bool>('offstage', offstage));
}
@override
SingleChildRenderObjectElement createElement() => _OffstageElement(this);
}
class _OffstageElement extends SingleChildRenderObjectElement {
_OffstageElement(Offstage super.widget);
@override
void debugVisitOnstageChildren(ElementVisitor visitor) {
if (!(widget as Offstage).offstage) {
super.debugVisitOnstageChildren(visitor);
}
}
}
/// A widget that attempts to size the child to a specific aspect ratio.
///
/// The aspect ratio is expressed as a ratio of width to height. For example, a
/// 16:9 width:height aspect ratio would have a value of 16.0/9.0.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=XcnP3_mO_Ms}
///
/// The [AspectRatio] widget uses a finite iterative process to compute the
/// appropriate constraints for the child, and then lays the child out a single
/// time with those constraints. This iterative process is efficient and does
/// not require multiple layout passes.
///
/// The widget first tries the largest width permitted by the layout
/// constraints, and determines the height of the widget by applying the given
/// aspect ratio to the width, expressed as a ratio of width to height.
///
/// If the maximum width is infinite, the initial width is determined
/// by applying the aspect ratio to the maximum height instead.
///
/// The widget then examines if the computed dimensions are compatible with the
/// parent's constraints; if not, the dimensions are recomputed a second time,
/// taking those constraints into account.
///
/// If the widget does not find a feasible size after consulting each
/// constraint, the widget will eventually select a size for the child that
/// meets the layout constraints but fails to meet the aspect ratio constraints.
///
/// {@tool dartpad}
/// This examples shows how [AspectRatio] sets the width when its parent's width
/// constraint is infinite. Since the parent's allowed height is a fixed value,
/// the actual width is determined via the given [aspectRatio].
///
/// In this example, the height is fixed at 100.0 and the aspect ratio is set to
/// 16 / 9, making the width 100.0 / 9 * 16.
///
/// ** See code in examples/api/lib/widgets/basic/aspect_ratio.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This second example uses an aspect ratio of 2.0, and layout constraints that
/// require the width to be between 0.0 and 100.0, and the height to be between
/// 0.0 and 100.0. The widget selects a width of 100.0 (the biggest allowed) and
/// a height of 50.0 (to match the aspect ratio).
///
/// ** See code in examples/api/lib/widgets/basic/aspect_ratio.1.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This third example is similar to the second, but with the aspect ratio set
/// to 0.5. The widget still selects a width of 100.0 (the biggest allowed), and
/// attempts to use a height of 200.0. Unfortunately, that violates the
/// constraints because the child can be at most 100.0 pixels tall. The widget
/// will then take that value and apply the aspect ratio again to obtain a width
/// of 50.0. That width is permitted by the constraints and the child receives a
/// width of 50.0 and a height of 100.0. If the width were not permitted, the
/// widget would continue iterating through the constraints.
///
/// ** See code in examples/api/lib/widgets/basic/aspect_ratio.2.dart **
/// {@end-tool}
///
/// ## Setting the aspect ratio in unconstrained situations
///
/// When using a widget such as [FittedBox], the constraints are unbounded. This
/// results in [AspectRatio] being unable to find a suitable set of constraints
/// to apply. In that situation, consider explicitly setting a size using
/// [SizedBox] instead of setting the aspect ratio using [AspectRatio]. The size
/// is then scaled appropriately by the [FittedBox].
///
/// See also:
///
/// * [Align], a widget that aligns its child within itself and optionally
/// sizes itself based on the child's size.
/// * [ConstrainedBox], a widget that imposes additional constraints on its
/// child.
/// * [UnconstrainedBox], a container that tries to let its child draw without
/// constraints.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class AspectRatio extends SingleChildRenderObjectWidget {
/// Creates a widget with a specific aspect ratio.
///
/// The [aspectRatio] argument must be a finite number greater than zero.
const AspectRatio({
super.key,
required this.aspectRatio,
super.child,
}) : assert(aspectRatio > 0.0);
/// The aspect ratio to attempt to use.
///
/// The aspect ratio is expressed as a ratio of width to height. For example,
/// a 16:9 width:height aspect ratio would have a value of 16.0/9.0.
final double aspectRatio;
@override
RenderAspectRatio createRenderObject(BuildContext context) => RenderAspectRatio(aspectRatio: aspectRatio);
@override
void updateRenderObject(BuildContext context, RenderAspectRatio renderObject) {
renderObject.aspectRatio = aspectRatio;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('aspectRatio', aspectRatio));
}
}
/// A widget that sizes its child to the child's maximum intrinsic width.
///
/// This class is useful, for example, when unlimited width is available and
/// you would like a child that would otherwise attempt to expand infinitely to
/// instead size itself to a more reasonable width.
///
/// The constraints that this widget passes to its child will adhere to the
/// parent's constraints, so if the constraints are not large enough to satisfy
/// the child's maximum intrinsic width, then the child will get less width
/// than it otherwise would. Likewise, if the minimum width constraint is
/// larger than the child's maximum intrinsic width, the child will be given
/// more width than it otherwise would.
///
/// If [stepWidth] is non-null, the child's width will be snapped to a multiple
/// of the [stepWidth]. Similarly, if [stepHeight] is non-null, the child's
/// height will be snapped to a multiple of the [stepHeight].
///
/// This class is relatively expensive, because it adds a speculative layout
/// pass before the final layout phase. Avoid using it where possible. In the
/// worst case, this widget can result in a layout that is O(N²) in the depth of
/// the tree.
///
/// See also:
///
/// * [Align], a widget that aligns its child within itself. This can be used
/// to loosen the constraints passed to the [RenderIntrinsicWidth],
/// allowing the [RenderIntrinsicWidth]'s child to be smaller than that of
/// its parent.
/// * [Row], which when used with [CrossAxisAlignment.stretch] can be used
/// to loosen just the width constraints that are passed to the
/// [RenderIntrinsicWidth], allowing the [RenderIntrinsicWidth]'s child's
/// width to be smaller than that of its parent.
/// * [The catalog of layout widgets](https://flutter.dev/widgets/layout/).
class IntrinsicWidth extends SingleChildRenderObjectWidget {
/// Creates a widget that sizes its child to the child's intrinsic width.
///
/// This class is relatively expensive. Avoid using it where possible.
const IntrinsicWidth({ super.key, this.stepWidth, this.stepHeight, super.child })
: assert(stepWidth == null || stepWidth >= 0.0),
assert(stepHeight == null || stepHeight >= 0.0);
/// If non-null, force the child's width to be a multiple of this value.
///
/// If null or 0.0 the child's width will be the same as its maximum
/// intrinsic width.
///
/// This value must not be negative.
///
/// See also:
///
/// * [RenderBox.getMaxIntrinsicWidth], which defines a widget's max
/// intrinsic width in general.
final double? stepWidth;
/// If non-null, force the child's height to be a multiple of this value.
///
/// If null or 0.0 the child's height will not be constrained.
///
/// This value must not be negative.
final double? stepHeight;
double? get _stepWidth => stepWidth == 0.0 ? null : stepWidth;
double? get _stepHeight => stepHeight == 0.0 ? null : stepHeight;
@override
RenderIntrinsicWidth createRenderObject(BuildContext context) {
return RenderIntrinsicWidth(stepWidth: _stepWidth, stepHeight: _stepHeight);
}
@override
void updateRenderObject(BuildContext context, RenderIntrinsicWidth renderObject) {
renderObject
..stepWidth = _stepWidth
..stepHeight = _stepHeight;
}
}
/// A widget that sizes its child to the child's intrinsic height.
///
/// This class is useful, for example, when unlimited height is available and
/// you would like a child that would otherwise attempt to expand infinitely to
/// instead size itself to a more reasonable height.
///
/// The constraints that this widget passes to its child will adhere to the
/// parent's constraints, so if the constraints are not large enough to satisfy
/// the child's maximum intrinsic height, then the child will get less height
/// than it otherwise would. Likewise, if the minimum height constraint is
/// larger than the child's maximum intrinsic height, the child will be given
/// more height than it otherwise would.
///
/// This class is relatively expensive, because it adds a speculative layout
/// pass before the final layout phase. Avoid using it where possible. In the
/// worst case, this widget can result in a layout that is O(N²) in the depth of
/// the tree.
///
/// See also:
///
/// * [Align], a widget that aligns its child within itself. This can be used
/// to loosen the constraints passed to the [RenderIntrinsicHeight],
/// allowing the [RenderIntrinsicHeight]'s child to be smaller than that of
/// its parent.
/// * [Column], which when used with [CrossAxisAlignment.stretch] can be used
/// to loosen just the height constraints that are passed to the
/// [RenderIntrinsicHeight], allowing the [RenderIntrinsicHeight]'s child's
/// height to be smaller than that of its parent.
/// * [The catalog of layout widgets](https://flutter.dev/widgets/layout/).
class IntrinsicHeight extends SingleChildRenderObjectWidget {
/// Creates a widget that sizes its child to the child's intrinsic height.
///
/// This class is relatively expensive. Avoid using it where possible.
const IntrinsicHeight({ super.key, super.child });
@override
RenderIntrinsicHeight createRenderObject(BuildContext context) => RenderIntrinsicHeight();
}
/// A widget that positions its child according to the child's baseline.
///
/// This widget shifts the child down such that the child's baseline (or the
/// bottom of the child, if the child has no baseline) is [baseline]
/// logical pixels below the top of this box, then sizes this box to
/// contain the child. If [baseline] is less than the distance from
/// the top of the child to the baseline of the child, then the child
/// is top-aligned instead.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=8ZaFk0yvNlI}
///
/// See also:
///
/// * [Align], a widget that aligns its child within itself and optionally
/// sizes itself based on the child's size.
/// * [Center], a widget that centers its child within itself.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class Baseline extends SingleChildRenderObjectWidget {
/// Creates a widget that positions its child according to the child's baseline.
const Baseline({
super.key,
required this.baseline,
required this.baselineType,
super.child,
});
/// The number of logical pixels from the top of this box at which to position
/// the child's baseline.
final double baseline;
/// The type of baseline to use for positioning the child.
final TextBaseline baselineType;
@override
RenderBaseline createRenderObject(BuildContext context) {
return RenderBaseline(baseline: baseline, baselineType: baselineType);
}
@override
void updateRenderObject(BuildContext context, RenderBaseline renderObject) {
renderObject
..baseline = baseline
..baselineType = baselineType;
}
}
/// A widget that causes the parent to ignore the [child] for the purposes
/// of baseline alignment.
///
/// See also:
///
/// * [Baseline], a widget that positions a child relative to a baseline.
class IgnoreBaseline extends SingleChildRenderObjectWidget {
/// Creates a widget that ignores the child for baseline alignment purposes.
const IgnoreBaseline({
super.key,
super.child,
});
@override
RenderIgnoreBaseline createRenderObject(BuildContext context) {
return RenderIgnoreBaseline();
}
}
// SLIVERS
/// A sliver that contains a single box widget.
///
/// Slivers are special-purpose widgets that can be combined using a
/// [CustomScrollView] to create custom scroll effects. A [SliverToBoxAdapter]
/// is a basic sliver that creates a bridge back to one of the usual box-based
/// widgets.
///
/// _To learn more about slivers, see [CustomScrollView.slivers]._
///
/// Rather than using multiple [SliverToBoxAdapter] widgets to display multiple
/// box widgets in a [CustomScrollView], consider using [SliverList],
/// [SliverFixedExtentList], [SliverPrototypeExtentList], or [SliverGrid],
/// which are more efficient because they instantiate only those children that
/// are actually visible through the scroll view's viewport.
///
/// See also:
///
/// * [CustomScrollView], which displays a scrollable list of slivers.
/// * [SliverList], which displays multiple box widgets in a linear array.
/// * [SliverFixedExtentList], which displays multiple box widgets with the
/// same main-axis extent in a linear array.
/// * [SliverPrototypeExtentList], which displays multiple box widgets with the
/// same main-axis extent as a prototype item, in a linear array.
/// * [SliverGrid], which displays multiple box widgets in arbitrary positions.
class SliverToBoxAdapter extends SingleChildRenderObjectWidget {
/// Creates a sliver that contains a single box widget.
const SliverToBoxAdapter({
super.key,
super.child,
});
@override
RenderSliverToBoxAdapter createRenderObject(BuildContext context) => RenderSliverToBoxAdapter();
}
/// A sliver that applies padding on each side of another sliver.
///
/// Slivers are special-purpose widgets that can be combined using a
/// [CustomScrollView] to create custom scroll effects. A [SliverPadding]
/// is a basic sliver that insets another sliver by applying padding on each
/// side.
///
/// {@macro flutter.rendering.RenderSliverEdgeInsetsPadding}
///
/// See also:
///
/// * [CustomScrollView], which displays a scrollable list of slivers.
/// * [Padding], the box version of this widget.
class SliverPadding extends SingleChildRenderObjectWidget {
/// Creates a sliver that applies padding on each side of another sliver.
const SliverPadding({
super.key,
required this.padding,
Widget? sliver,
}) : super(child: sliver);
/// The amount of space by which to inset the child sliver.
final EdgeInsetsGeometry padding;
@override
RenderSliverPadding createRenderObject(BuildContext context) {
return RenderSliverPadding(
padding: padding,
textDirection: Directionality.of(context),
);
}
@override
void updateRenderObject(BuildContext context, RenderSliverPadding renderObject) {
renderObject
..padding = padding
..textDirection = Directionality.of(context);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<EdgeInsetsGeometry>('padding', padding));
}
}
// LAYOUT NODES
/// Returns the [AxisDirection] in the given [Axis] in the current
/// [Directionality] (or the reverse if `reverse` is true).
///
/// If `axis` is [Axis.vertical], this function returns [AxisDirection.down]
/// unless `reverse` is true, in which case this function returns
/// [AxisDirection.up].
///
/// If `axis` is [Axis.horizontal], this function checks the current
/// [Directionality]. If the current [Directionality] is right-to-left, then
/// this function returns [AxisDirection.left] (unless `reverse` is true, in
/// which case it returns [AxisDirection.right]). Similarly, if the current
/// [Directionality] is left-to-right, then this function returns
/// [AxisDirection.right] (unless `reverse` is true, in which case it returns
/// [AxisDirection.left]).
///
/// This function is used by a number of scrolling widgets (e.g., [ListView],
/// [GridView], [PageView], and [SingleChildScrollView]) as well as [ListBody]
/// to translate their [Axis] and `reverse` properties into a concrete
/// [AxisDirection].
AxisDirection getAxisDirectionFromAxisReverseAndDirectionality(
BuildContext context,
Axis axis,
bool reverse,
) {
switch (axis) {
case Axis.horizontal:
assert(debugCheckHasDirectionality(context));
final TextDirection textDirection = Directionality.of(context);
final AxisDirection axisDirection = textDirectionToAxisDirection(textDirection);
return reverse ? flipAxisDirection(axisDirection) : axisDirection;
case Axis.vertical:
return reverse ? AxisDirection.up : AxisDirection.down;
}
}
/// A widget that arranges its children sequentially along a given axis, forcing
/// them to the dimension of the parent in the other axis.
///
/// This widget is rarely used directly. Instead, consider using [ListView],
/// which combines a similar layout algorithm with scrolling behavior, or
/// [Column], which gives you more flexible control over the layout of a
/// vertical set of boxes.
///
/// See also:
///
/// * [RenderListBody], which implements this layout algorithm and the
/// documentation for which describes some of its subtleties.
/// * [SingleChildScrollView], which is sometimes used with [ListBody] to
/// make the contents scrollable.
/// * [Column] and [Row], which implement a more elaborate version of
/// this layout algorithm (at the cost of being slightly less efficient).
/// * [ListView], which implements an efficient scrolling version of this
/// layout algorithm.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class ListBody extends MultiChildRenderObjectWidget {
/// Creates a layout widget that arranges its children sequentially along a
/// given axis.
///
/// By default, the [mainAxis] is [Axis.vertical].
const ListBody({
super.key,
this.mainAxis = Axis.vertical,
this.reverse = false,
super.children,
});
/// The direction to use as the main axis.
final Axis mainAxis;
/// Whether the list body positions children in the reading direction.
///
/// For example, if the reading direction is left-to-right and
/// [mainAxis] is [Axis.horizontal], then the list body positions children
/// from left to right when [reverse] is false and from right to left when
/// [reverse] is true.
///
/// Similarly, if [mainAxis] is [Axis.vertical], then the list body positions
/// from top to bottom when [reverse] is false and from bottom to top when
/// [reverse] is true.
///
/// Defaults to false.
final bool reverse;
AxisDirection _getDirection(BuildContext context) {
return getAxisDirectionFromAxisReverseAndDirectionality(context, mainAxis, reverse);
}
@override
RenderListBody createRenderObject(BuildContext context) {
return RenderListBody(axisDirection: _getDirection(context));
}
@override
void updateRenderObject(BuildContext context, RenderListBody renderObject) {
renderObject.axisDirection = _getDirection(context);
}
}
/// A widget that positions its children relative to the edges of its box.
///
/// This class is useful if you want to overlap several children in a simple
/// way, for example having some text and an image, overlaid with a gradient and
/// a button attached to the bottom.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=liEGSeD3Zt8}
///
/// Each child of a [Stack] widget is either _positioned_ or _non-positioned_.
/// Positioned children are those wrapped in a [Positioned] widget that has at
/// least one non-null property. The stack sizes itself to contain all the
/// non-positioned children, which are positioned according to [alignment]
/// (which defaults to the top-left corner in left-to-right environments and the
/// top-right corner in right-to-left environments). The positioned children are
/// then placed relative to the stack according to their top, right, bottom, and
/// left properties.
///
/// The stack paints its children in order with the first child being at the
/// bottom. If you want to change the order in which the children paint, you
/// can rebuild the stack with the children in the new order. If you reorder
/// the children in this way, consider giving the children non-null keys.
/// These keys will cause the framework to move the underlying objects for
/// the children to their new locations rather than recreate them at their
/// new location.
///
/// For more details about the stack layout algorithm, see [RenderStack].
///
/// If you want to lay a number of children out in a particular pattern, or if
/// you want to make a custom layout manager, you probably want to use
/// [CustomMultiChildLayout] instead. In particular, when using a [Stack] you
/// can't position children relative to their size or the stack's own size.
///
/// {@tool snippet}
///
/// Using a [Stack] you can position widgets over one another.
///
/// 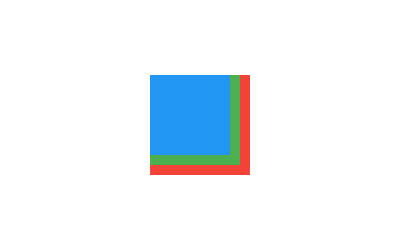
///
/// ```dart
/// Stack(
/// children: <Widget>[
/// Container(
/// width: 100,
/// height: 100,
/// color: Colors.red,
/// ),
/// Container(
/// width: 90,
/// height: 90,
/// color: Colors.green,
/// ),
/// Container(
/// width: 80,
/// height: 80,
/// color: Colors.blue,
/// ),
/// ],
/// )
/// ```
/// {@end-tool}
///
/// {@tool snippet}
///
/// This example shows how [Stack] can be used to enhance text visibility
/// by adding gradient backdrops.
///
/// 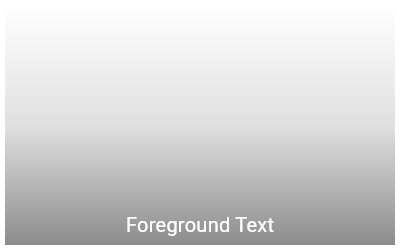
///
/// ```dart
/// SizedBox(
/// width: 250,
/// height: 250,
/// child: Stack(
/// children: <Widget>[
/// Container(
/// width: 250,
/// height: 250,
/// color: Colors.white,
/// ),
/// Container(
/// padding: const EdgeInsets.all(5.0),
/// alignment: Alignment.bottomCenter,
/// decoration: BoxDecoration(
/// gradient: LinearGradient(
/// begin: Alignment.topCenter,
/// end: Alignment.bottomCenter,
/// colors: <Color>[
/// Colors.black.withAlpha(0),
/// Colors.black12,
/// Colors.black45
/// ],
/// ),
/// ),
/// child: const Text(
/// 'Foreground Text',
/// style: TextStyle(color: Colors.white, fontSize: 20.0),
/// ),
/// ),
/// ],
/// ),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [Align], which sizes itself based on its child's size and positions
/// the child according to an [Alignment] value.
/// * [CustomSingleChildLayout], which uses a delegate to control the layout of
/// a single child.
/// * [CustomMultiChildLayout], which uses a delegate to position multiple
/// children.
/// * [Flow], which provides paint-time control of its children using transform
/// matrices.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class Stack extends MultiChildRenderObjectWidget {
/// Creates a stack layout widget.
///
/// By default, the non-positioned children of the stack are aligned by their
/// top left corners.
const Stack({
super.key,
this.alignment = AlignmentDirectional.topStart,
this.textDirection,
this.fit = StackFit.loose,
this.clipBehavior = Clip.hardEdge,
super.children,
});
/// How to align the non-positioned and partially-positioned children in the
/// stack.
///
/// The non-positioned children are placed relative to each other such that
/// the points determined by [alignment] are co-located. For example, if the
/// [alignment] is [Alignment.topLeft], then the top left corner of
/// each non-positioned child will be located at the same global coordinate.
///
/// Partially-positioned children, those that do not specify an alignment in a
/// particular axis (e.g. that have neither `top` nor `bottom` set), use the
/// alignment to determine how they should be positioned in that
/// under-specified axis.
///
/// Defaults to [AlignmentDirectional.topStart].
///
/// See also:
///
/// * [Alignment], a class with convenient constants typically used to
/// specify an [AlignmentGeometry].
/// * [AlignmentDirectional], like [Alignment] for specifying alignments
/// relative to text direction.
final AlignmentGeometry alignment;
/// The text direction with which to resolve [alignment].
///
/// Defaults to the ambient [Directionality].
final TextDirection? textDirection;
/// How to size the non-positioned children in the stack.
///
/// The constraints passed into the [Stack] from its parent are either
/// loosened ([StackFit.loose]) or tightened to their biggest size
/// ([StackFit.expand]).
final StackFit fit;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Stacks only clip children whose _geometry_ overflows the stack. A child
/// that paints outside its bounds (e.g. a box with a shadow) will not be
/// clipped, regardless of the value of this property. Similarly, a child that
/// itself has a descendant that overflows the stack will not be clipped, as
/// only the geometry of the stack's direct children are considered.
/// [Transform] is an example of a widget that can cause its children to paint
/// outside its geometry.
///
/// To clip children whose geometry does not overflow the stack, consider
/// using a [ClipRect] widget.
///
/// Defaults to [Clip.hardEdge].
final Clip clipBehavior;
bool _debugCheckHasDirectionality(BuildContext context) {
if (alignment is AlignmentDirectional && textDirection == null) {
assert(debugCheckHasDirectionality(
context,
why: "to resolve the 'alignment' argument",
hint: alignment == AlignmentDirectional.topStart ? "The default value for 'alignment' is AlignmentDirectional.topStart, which requires a text direction." : null,
alternative: "Instead of providing a Directionality widget, another solution would be passing a non-directional 'alignment', or an explicit 'textDirection', to the $runtimeType.",
));
}
return true;
}
@override
RenderStack createRenderObject(BuildContext context) {
assert(_debugCheckHasDirectionality(context));
return RenderStack(
alignment: alignment,
textDirection: textDirection ?? Directionality.maybeOf(context),
fit: fit,
clipBehavior: clipBehavior,
);
}
@override
void updateRenderObject(BuildContext context, RenderStack renderObject) {
assert(_debugCheckHasDirectionality(context));
renderObject
..alignment = alignment
..textDirection = textDirection ?? Directionality.maybeOf(context)
..fit = fit
..clipBehavior = clipBehavior;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<AlignmentGeometry>('alignment', alignment));
properties.add(EnumProperty<TextDirection>('textDirection', textDirection, defaultValue: null));
properties.add(EnumProperty<StackFit>('fit', fit));
properties.add(EnumProperty<Clip>('clipBehavior', clipBehavior, defaultValue: Clip.hardEdge));
}
}
/// A [Stack] that shows a single child from a list of children.
///
/// The displayed child is the one with the given [index]. The stack is
/// always as big as the largest child.
///
/// If value is null, then nothing is displayed.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=_O0PPD1Xfbk}
///
/// {@tool dartpad}
/// This example shows a [IndexedStack] widget being used to lay out one card
/// at a time from a series of cards, each keeping their respective states.
///
/// ** See code in examples/api/lib/widgets/basic/indexed_stack.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [Stack], for more details about stacks.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class IndexedStack extends StatelessWidget {
/// Creates a [Stack] widget that paints a single child.
const IndexedStack({
super.key,
this.alignment = AlignmentDirectional.topStart,
this.textDirection,
this.clipBehavior = Clip.hardEdge,
this.sizing = StackFit.loose,
this.index = 0,
this.children = const <Widget>[],
});
/// How to align the non-positioned and partially-positioned children in the
/// stack.
///
/// Defaults to [AlignmentDirectional.topStart].
///
/// See [Stack.alignment] for more information.
final AlignmentGeometry alignment;
/// The text direction with which to resolve [alignment].
///
/// Defaults to the ambient [Directionality].
final TextDirection? textDirection;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.hardEdge].
final Clip clipBehavior;
/// How to size the non-positioned children in the stack.
///
/// Defaults to [StackFit.loose].
///
/// See [Stack.fit] for more information.
final StackFit sizing;
/// The index of the child to show.
///
/// If this is null, none of the children will be shown.
final int? index;
/// The child widgets of the stack.
///
/// Only the child at index [index] will be shown.
///
/// See [Stack.children] for more information.
final List<Widget> children;
@override
Widget build(BuildContext context) {
final List<Widget> wrappedChildren = List<Widget>.generate(children.length, (int i) {
return Visibility.maintain(
visible: i == index,
child: children[i],
);
});
return _RawIndexedStack(
alignment: alignment,
textDirection: textDirection,
clipBehavior: clipBehavior,
sizing: sizing,
index: index,
children: wrappedChildren,
);
}
}
/// The render object widget that backs [IndexedStack].
class _RawIndexedStack extends Stack {
/// Creates a [Stack] widget that paints a single child.
const _RawIndexedStack({
super.alignment,
super.textDirection,
super.clipBehavior,
StackFit sizing = StackFit.loose,
this.index = 0,
super.children,
}) : super(fit: sizing);
/// The index of the child to show.
final int? index;
@override
RenderIndexedStack createRenderObject(BuildContext context) {
assert(_debugCheckHasDirectionality(context));
return RenderIndexedStack(
index: index,
fit: fit,
clipBehavior: clipBehavior,
alignment: alignment,
textDirection: textDirection ?? Directionality.maybeOf(context),
);
}
@override
void updateRenderObject(BuildContext context, RenderIndexedStack renderObject) {
assert(_debugCheckHasDirectionality(context));
renderObject
..index = index
..fit = fit
..clipBehavior = clipBehavior
..alignment = alignment
..textDirection = textDirection ?? Directionality.maybeOf(context);
}
@override
MultiChildRenderObjectElement createElement() {
return _IndexedStackElement(this);
}
}
class _IndexedStackElement extends MultiChildRenderObjectElement {
_IndexedStackElement(_RawIndexedStack super.widget);
@override
_RawIndexedStack get widget => super.widget as _RawIndexedStack;
@override
void debugVisitOnstageChildren(ElementVisitor visitor) {
final int? index = widget.index;
// If the index is null, no child is onstage. Otherwise, only the child at
// the selected index is.
if (index != null && children.isNotEmpty) {
visitor(children.elementAt(index));
}
}
}
/// A widget that controls where a child of a [Stack] is positioned.
///
/// A [Positioned] widget must be a descendant of a [Stack], and the path from
/// the [Positioned] widget to its enclosing [Stack] must contain only
/// [StatelessWidget]s or [StatefulWidget]s (not other kinds of widgets, like
/// [RenderObjectWidget]s).
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=EgtPleVwxBQ}
///
/// If a widget is wrapped in a [Positioned], then it is a _positioned_ widget
/// in its [Stack]. If the [top] property is non-null, the top edge of this child
/// will be positioned [top] layout units from the top of the stack widget. The
/// [right], [bottom], and [left] properties work analogously.
///
/// If both the [top] and [bottom] properties are non-null, then the child will
/// be forced to have exactly the height required to satisfy both constraints.
/// Similarly, setting the [right] and [left] properties to non-null values will
/// force the child to have a particular width. Alternatively the [width] and
/// [height] properties can be used to give the dimensions, with one
/// corresponding position property (e.g. [top] and [height]).
///
/// If all three values on a particular axis are null, then the
/// [Stack.alignment] property is used to position the child.
///
/// If all six values are null, the child is a non-positioned child. The [Stack]
/// uses only the non-positioned children to size itself.
///
/// See also:
///
/// * [AnimatedPositioned], which automatically transitions the child's
/// position over a given duration whenever the given position changes.
/// * [PositionedTransition], which takes a provided [Animation] to transition
/// changes in the child's position over a given duration.
/// * [PositionedDirectional], which adapts to the ambient [Directionality].
class Positioned extends ParentDataWidget<StackParentData> {
/// Creates a widget that controls where a child of a [Stack] is positioned.
///
/// Only two out of the three horizontal values ([left], [right],
/// [width]), and only two out of the three vertical values ([top],
/// [bottom], [height]), can be set. In each case, at least one of
/// the three must be null.
///
/// See also:
///
/// * [Positioned.directional], which specifies the widget's horizontal
/// position using `start` and `end` rather than `left` and `right`.
/// * [PositionedDirectional], which is similar to [Positioned.directional]
/// but adapts to the ambient [Directionality].
const Positioned({
super.key,
this.left,
this.top,
this.right,
this.bottom,
this.width,
this.height,
required super.child,
}) : assert(left == null || right == null || width == null),
assert(top == null || bottom == null || height == null);
/// Creates a Positioned object with the values from the given [Rect].
///
/// This sets the [left], [top], [width], and [height] properties
/// from the given [Rect]. The [right] and [bottom] properties are
/// set to null.
Positioned.fromRect({
super.key,
required Rect rect,
required super.child,
}) : left = rect.left,
top = rect.top,
width = rect.width,
height = rect.height,
right = null,
bottom = null;
/// Creates a Positioned object with the values from the given [RelativeRect].
///
/// This sets the [left], [top], [right], and [bottom] properties from the
/// given [RelativeRect]. The [height] and [width] properties are set to null.
Positioned.fromRelativeRect({
super.key,
required RelativeRect rect,
required super.child,
}) : left = rect.left,
top = rect.top,
right = rect.right,
bottom = rect.bottom,
width = null,
height = null;
/// Creates a Positioned object with [left], [top], [right], and [bottom] set
/// to 0.0 unless a value for them is passed.
const Positioned.fill({
super.key,
this.left = 0.0,
this.top = 0.0,
this.right = 0.0,
this.bottom = 0.0,
required super.child,
}) : width = null,
height = null;
/// Creates a widget that controls where a child of a [Stack] is positioned.
///
/// Only two out of the three horizontal values (`start`, `end`,
/// [width]), and only two out of the three vertical values ([top],
/// [bottom], [height]), can be set. In each case, at least one of
/// the three must be null.
///
/// If `textDirection` is [TextDirection.rtl], then the `start` argument is
/// used for the [right] property and the `end` argument is used for the
/// [left] property. Otherwise, if `textDirection` is [TextDirection.ltr],
/// then the `start` argument is used for the [left] property and the `end`
/// argument is used for the [right] property.
///
/// See also:
///
/// * [PositionedDirectional], which adapts to the ambient [Directionality].
factory Positioned.directional({
Key? key,
required TextDirection textDirection,
double? start,
double? top,
double? end,
double? bottom,
double? width,
double? height,
required Widget child,
}) {
final (double? left, double? right) = switch (textDirection) {
TextDirection.rtl => (end, start),
TextDirection.ltr => (start, end),
};
return Positioned(
key: key,
left: left,
top: top,
right: right,
bottom: bottom,
width: width,
height: height,
child: child,
);
}
/// The distance that the child's left edge is inset from the left of the stack.
///
/// Only two out of the three horizontal values ([left], [right], [width]) can be
/// set. The third must be null.
///
/// If all three are null, the [Stack.alignment] is used to position the child
/// horizontally.
final double? left;
/// The distance that the child's top edge is inset from the top of the stack.
///
/// Only two out of the three vertical values ([top], [bottom], [height]) can be
/// set. The third must be null.
///
/// If all three are null, the [Stack.alignment] is used to position the child
/// vertically.
final double? top;
/// The distance that the child's right edge is inset from the right of the stack.
///
/// Only two out of the three horizontal values ([left], [right], [width]) can be
/// set. The third must be null.
///
/// If all three are null, the [Stack.alignment] is used to position the child
/// horizontally.
final double? right;
/// The distance that the child's bottom edge is inset from the bottom of the stack.
///
/// Only two out of the three vertical values ([top], [bottom], [height]) can be
/// set. The third must be null.
///
/// If all three are null, the [Stack.alignment] is used to position the child
/// vertically.
final double? bottom;
/// The child's width.
///
/// Only two out of the three horizontal values ([left], [right], [width]) can be
/// set. The third must be null.
///
/// If all three are null, the [Stack.alignment] is used to position the child
/// horizontally.
final double? width;
/// The child's height.
///
/// Only two out of the three vertical values ([top], [bottom], [height]) can be
/// set. The third must be null.
///
/// If all three are null, the [Stack.alignment] is used to position the child
/// vertically.
final double? height;
@override
void applyParentData(RenderObject renderObject) {
assert(renderObject.parentData is StackParentData);
final StackParentData parentData = renderObject.parentData! as StackParentData;
bool needsLayout = false;
if (parentData.left != left) {
parentData.left = left;
needsLayout = true;
}
if (parentData.top != top) {
parentData.top = top;
needsLayout = true;
}
if (parentData.right != right) {
parentData.right = right;
needsLayout = true;
}
if (parentData.bottom != bottom) {
parentData.bottom = bottom;
needsLayout = true;
}
if (parentData.width != width) {
parentData.width = width;
needsLayout = true;
}
if (parentData.height != height) {
parentData.height = height;
needsLayout = true;
}
if (needsLayout) {
final RenderObject? targetParent = renderObject.parent;
if (targetParent is RenderObject) {
targetParent.markNeedsLayout();
}
}
}
@override
Type get debugTypicalAncestorWidgetClass => Stack;
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('left', left, defaultValue: null));
properties.add(DoubleProperty('top', top, defaultValue: null));
properties.add(DoubleProperty('right', right, defaultValue: null));
properties.add(DoubleProperty('bottom', bottom, defaultValue: null));
properties.add(DoubleProperty('width', width, defaultValue: null));
properties.add(DoubleProperty('height', height, defaultValue: null));
}
}
/// A widget that controls where a child of a [Stack] is positioned without
/// committing to a specific [TextDirection].
///
/// The ambient [Directionality] is used to determine whether [start] is to the
/// left or to the right.
///
/// A [PositionedDirectional] widget must be a descendant of a [Stack], and the
/// path from the [PositionedDirectional] widget to its enclosing [Stack] must
/// contain only [StatelessWidget]s or [StatefulWidget]s (not other kinds of
/// widgets, like [RenderObjectWidget]s).
///
/// If a widget is wrapped in a [PositionedDirectional], then it is a
/// _positioned_ widget in its [Stack]. If the [top] property is non-null, the
/// top edge of this child/ will be positioned [top] layout units from the top
/// of the stack widget. The [start], [bottom], and [end] properties work
/// analogously.
///
/// If both the [top] and [bottom] properties are non-null, then the child will
/// be forced to have exactly the height required to satisfy both constraints.
/// Similarly, setting the [start] and [end] properties to non-null values will
/// force the child to have a particular width. Alternatively the [width] and
/// [height] properties can be used to give the dimensions, with one
/// corresponding position property (e.g. [top] and [height]).
///
/// See also:
///
/// * [Positioned], which specifies the widget's position visually.
/// * [Positioned.directional], which also specifies the widget's horizontal
/// position using [start] and [end] but has an explicit [TextDirection].
/// * [AnimatedPositionedDirectional], which automatically transitions
/// the child's position over a given duration whenever the given position
/// changes.
class PositionedDirectional extends StatelessWidget {
/// Creates a widget that controls where a child of a [Stack] is positioned.
///
/// Only two out of the three horizontal values (`start`, `end`,
/// [width]), and only two out of the three vertical values ([top],
/// [bottom], [height]), can be set. In each case, at least one of
/// the three must be null.
///
/// See also:
///
/// * [Positioned.directional], which also specifies the widget's horizontal
/// position using [start] and [end] but has an explicit [TextDirection].
const PositionedDirectional({
super.key,
this.start,
this.top,
this.end,
this.bottom,
this.width,
this.height,
required this.child,
});
/// The distance that the child's leading edge is inset from the leading edge
/// of the stack.
///
/// Only two out of the three horizontal values ([start], [end], [width]) can be
/// set. The third must be null.
final double? start;
/// The distance that the child's top edge is inset from the top of the stack.
///
/// Only two out of the three vertical values ([top], [bottom], [height]) can be
/// set. The third must be null.
final double? top;
/// The distance that the child's trailing edge is inset from the trailing
/// edge of the stack.
///
/// Only two out of the three horizontal values ([start], [end], [width]) can be
/// set. The third must be null.
final double? end;
/// The distance that the child's bottom edge is inset from the bottom of the stack.
///
/// Only two out of the three vertical values ([top], [bottom], [height]) can be
/// set. The third must be null.
final double? bottom;
/// The child's width.
///
/// Only two out of the three horizontal values ([start], [end], [width]) can be
/// set. The third must be null.
final double? width;
/// The child's height.
///
/// Only two out of the three vertical values ([top], [bottom], [height]) can be
/// set. The third must be null.
final double? height;
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
@override
Widget build(BuildContext context) {
return Positioned.directional(
textDirection: Directionality.of(context),
start: start,
top: top,
end: end,
bottom: bottom,
width: width,
height: height,
child: child,
);
}
}
/// A widget that displays its children in a one-dimensional array.
///
/// The [Flex] widget allows you to control the axis along which the children are
/// placed (horizontal or vertical). This is referred to as the _main axis_. If
/// you know the main axis in advance, then consider using a [Row] (if it's
/// horizontal) or [Column] (if it's vertical) instead, because that will be less
/// verbose.
///
/// To cause a child to expand to fill the available space in the [direction]
/// of this widget's main axis, wrap the child in an [Expanded] widget.
///
/// The [Flex] widget does not scroll (and in general it is considered an error
/// to have more children in a [Flex] than will fit in the available room). If
/// you have some widgets and want them to be able to scroll if there is
/// insufficient room, consider using a [ListView].
///
/// The [Flex] widget does not allow its children to wrap across multiple
/// horizontal or vertical runs. For a widget that allows its children to wrap,
/// consider using the [Wrap] widget instead of [Flex].
///
/// If you only have one child, then rather than using [Flex], [Row], or
/// [Column], consider using [Align] or [Center] to position the child.
///
/// ## Layout algorithm
///
/// _This section describes how a [Flex] is rendered by the framework._
/// _See [BoxConstraints] for an introduction to box layout models._
///
/// Layout for a [Flex] proceeds in six steps:
///
/// 1. Layout each child with a null or zero flex factor (e.g., those that are
/// not [Expanded]) with unbounded main axis constraints and the incoming
/// cross axis constraints. If the [crossAxisAlignment] is
/// [CrossAxisAlignment.stretch], instead use tight cross axis constraints
/// that match the incoming max extent in the cross axis.
/// 2. Divide the remaining main axis space among the children with non-zero
/// flex factors (e.g., those that are [Expanded]) according to their flex
/// factor. For example, a child with a flex factor of 2.0 will receive twice
/// the amount of main axis space as a child with a flex factor of 1.0.
/// 3. Layout each of the remaining children with the same cross axis
/// constraints as in step 1, but instead of using unbounded main axis
/// constraints, use max axis constraints based on the amount of space
/// allocated in step 2. Children with [Flexible.fit] properties that are
/// [FlexFit.tight] are given tight constraints (i.e., forced to fill the
/// allocated space), and children with [Flexible.fit] properties that are
/// [FlexFit.loose] are given loose constraints (i.e., not forced to fill the
/// allocated space).
/// 4. The cross axis extent of the [Flex] is the maximum cross axis extent of
/// the children (which will always satisfy the incoming constraints).
/// 5. The main axis extent of the [Flex] is determined by the [mainAxisSize]
/// property. If the [mainAxisSize] property is [MainAxisSize.max], then the
/// main axis extent of the [Flex] is the max extent of the incoming main
/// axis constraints. If the [mainAxisSize] property is [MainAxisSize.min],
/// then the main axis extent of the [Flex] is the sum of the main axis
/// extents of the children (subject to the incoming constraints).
/// 6. Determine the position for each child according to the
/// [mainAxisAlignment] and the [crossAxisAlignment]. For example, if the
/// [mainAxisAlignment] is [MainAxisAlignment.spaceBetween], any main axis
/// space that has not been allocated to children is divided evenly and
/// placed between the children.
///
/// See also:
///
/// * [Row], for a version of this widget that is always horizontal.
/// * [Column], for a version of this widget that is always vertical.
/// * [Expanded], to indicate children that should take all the remaining room.
/// * [Flexible], to indicate children that should share the remaining room.
/// * [Spacer], a widget that takes up space proportional to its flex value.
/// that may be sized smaller (leaving some remaining room unused).
/// * [Wrap], for a widget that allows its children to wrap over multiple _runs_.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class Flex extends MultiChildRenderObjectWidget {
/// Creates a flex layout.
///
/// The [direction] is required.
///
/// If [crossAxisAlignment] is [CrossAxisAlignment.baseline], then
/// [textBaseline] must not be null.
///
/// The [textDirection] argument defaults to the ambient [Directionality], if
/// any. If there is no ambient directionality, and a text direction is going
/// to be necessary to decide which direction to lay the children in or to
/// disambiguate `start` or `end` values for the main or cross axis
/// directions, the [textDirection] must not be null.
const Flex({
super.key,
required this.direction,
this.mainAxisAlignment = MainAxisAlignment.start,
this.mainAxisSize = MainAxisSize.max,
this.crossAxisAlignment = CrossAxisAlignment.center,
this.textDirection,
this.verticalDirection = VerticalDirection.down,
this.textBaseline, // NO DEFAULT: we don't know what the text's baseline should be
this.clipBehavior = Clip.none,
super.children,
}) : assert(!identical(crossAxisAlignment, CrossAxisAlignment.baseline) || textBaseline != null, 'textBaseline is required if you specify the crossAxisAlignment with CrossAxisAlignment.baseline');
// Cannot use == in the assert above instead of identical because of https://github.com/dart-lang/language/issues/1811.
/// The direction to use as the main axis.
///
/// If you know the axis in advance, then consider using a [Row] (if it's
/// horizontal) or [Column] (if it's vertical) instead of a [Flex], since that
/// will be less verbose. (For [Row] and [Column] this property is fixed to
/// the appropriate axis.)
final Axis direction;
/// How the children should be placed along the main axis.
///
/// For example, [MainAxisAlignment.start], the default, places the children
/// at the start (i.e., the left for a [Row] or the top for a [Column]) of the
/// main axis.
final MainAxisAlignment mainAxisAlignment;
/// How much space should be occupied in the main axis.
///
/// After allocating space to children, there might be some remaining free
/// space. This value controls whether to maximize or minimize the amount of
/// free space, subject to the incoming layout constraints.
///
/// If some children have a non-zero flex factors (and none have a fit of
/// [FlexFit.loose]), they will expand to consume all the available space and
/// there will be no remaining free space to maximize or minimize, making this
/// value irrelevant to the final layout.
final MainAxisSize mainAxisSize;
/// How the children should be placed along the cross axis.
///
/// For example, [CrossAxisAlignment.center], the default, centers the
/// children in the cross axis (e.g., horizontally for a [Column]).
///
/// When the cross axis is vertical (as for a [Row]) and the children
/// contain text, consider using [CrossAxisAlignment.baseline] instead.
/// This typically produces better visual results if the different children
/// have text with different font metrics, for example because they differ in
/// [TextStyle.fontSize] or other [TextStyle] properties, or because
/// they use different fonts due to being written in different scripts.
final CrossAxisAlignment crossAxisAlignment;
/// Determines the order to lay children out horizontally and how to interpret
/// `start` and `end` in the horizontal direction.
///
/// Defaults to the ambient [Directionality].
///
/// If [textDirection] is [TextDirection.rtl], then the direction in which
/// text flows starts from right to left. Otherwise, if [textDirection] is
/// [TextDirection.ltr], then the direction in which text flows starts from
/// left to right.
///
/// If the [direction] is [Axis.horizontal], this controls the order in which
/// the children are positioned (left-to-right or right-to-left), and the
/// meaning of the [mainAxisAlignment] property's [MainAxisAlignment.start] and
/// [MainAxisAlignment.end] values.
///
/// If the [direction] is [Axis.horizontal], and either the
/// [mainAxisAlignment] is either [MainAxisAlignment.start] or
/// [MainAxisAlignment.end], or there's more than one child, then the
/// [textDirection] (or the ambient [Directionality]) must not be null.
///
/// If the [direction] is [Axis.vertical], this controls the meaning of the
/// [crossAxisAlignment] property's [CrossAxisAlignment.start] and
/// [CrossAxisAlignment.end] values.
///
/// If the [direction] is [Axis.vertical], and the [crossAxisAlignment] is
/// either [CrossAxisAlignment.start] or [CrossAxisAlignment.end], then the
/// [textDirection] (or the ambient [Directionality]) must not be null.
final TextDirection? textDirection;
/// Determines the order to lay children out vertically and how to interpret
/// `start` and `end` in the vertical direction.
///
/// Defaults to [VerticalDirection.down].
///
/// If the [direction] is [Axis.vertical], this controls which order children
/// are painted in (down or up), the meaning of the [mainAxisAlignment]
/// property's [MainAxisAlignment.start] and [MainAxisAlignment.end] values.
///
/// If the [direction] is [Axis.vertical], and either the [mainAxisAlignment]
/// is either [MainAxisAlignment.start] or [MainAxisAlignment.end], or there's
/// more than one child, then the [verticalDirection] must not be null.
///
/// If the [direction] is [Axis.horizontal], this controls the meaning of the
/// [crossAxisAlignment] property's [CrossAxisAlignment.start] and
/// [CrossAxisAlignment.end] values.
///
/// If the [direction] is [Axis.horizontal], and the [crossAxisAlignment] is
/// either [CrossAxisAlignment.start] or [CrossAxisAlignment.end], then the
/// [verticalDirection] must not be null.
final VerticalDirection verticalDirection;
/// If aligning items according to their baseline, which baseline to use.
///
/// This must be set if using baseline alignment. There is no default because there is no
/// way for the framework to know the correct baseline _a priori_.
final TextBaseline? textBaseline;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.none].
final Clip clipBehavior;
bool get _needTextDirection {
switch (direction) {
case Axis.horizontal:
return true; // because it affects the layout order.
case Axis.vertical:
return crossAxisAlignment == CrossAxisAlignment.start
|| crossAxisAlignment == CrossAxisAlignment.end;
}
}
/// The value to pass to [RenderFlex.textDirection].
///
/// This value is derived from the [textDirection] property and the ambient
/// [Directionality]. The value is null if there is no need to specify the
/// text direction. In practice there's always a need to specify the direction
/// except for vertical flexes (e.g. [Column]s) whose [crossAxisAlignment] is
/// not dependent on the text direction (not `start` or `end`). In particular,
/// a [Row] always needs a text direction because the text direction controls
/// its layout order. (For [Column]s, the layout order is controlled by
/// [verticalDirection], which is always specified as it does not depend on an
/// inherited widget and defaults to [VerticalDirection.down].)
///
/// This method exists so that subclasses of [Flex] that create their own
/// render objects that are derived from [RenderFlex] can do so and still use
/// the logic for providing a text direction only when it is necessary.
@protected
TextDirection? getEffectiveTextDirection(BuildContext context) {
return textDirection ?? (_needTextDirection ? Directionality.maybeOf(context) : null);
}
@override
RenderFlex createRenderObject(BuildContext context) {
return RenderFlex(
direction: direction,
mainAxisAlignment: mainAxisAlignment,
mainAxisSize: mainAxisSize,
crossAxisAlignment: crossAxisAlignment,
textDirection: getEffectiveTextDirection(context),
verticalDirection: verticalDirection,
textBaseline: textBaseline,
clipBehavior: clipBehavior,
);
}
@override
void updateRenderObject(BuildContext context, covariant RenderFlex renderObject) {
renderObject
..direction = direction
..mainAxisAlignment = mainAxisAlignment
..mainAxisSize = mainAxisSize
..crossAxisAlignment = crossAxisAlignment
..textDirection = getEffectiveTextDirection(context)
..verticalDirection = verticalDirection
..textBaseline = textBaseline
..clipBehavior = clipBehavior;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<Axis>('direction', direction));
properties.add(EnumProperty<MainAxisAlignment>('mainAxisAlignment', mainAxisAlignment));
properties.add(EnumProperty<MainAxisSize>('mainAxisSize', mainAxisSize, defaultValue: MainAxisSize.max));
properties.add(EnumProperty<CrossAxisAlignment>('crossAxisAlignment', crossAxisAlignment));
properties.add(EnumProperty<TextDirection>('textDirection', textDirection, defaultValue: null));
properties.add(EnumProperty<VerticalDirection>('verticalDirection', verticalDirection, defaultValue: VerticalDirection.down));
properties.add(EnumProperty<TextBaseline>('textBaseline', textBaseline, defaultValue: null));
}
}
/// A widget that displays its children in a horizontal array.
///
/// To cause a child to expand to fill the available horizontal space, wrap the
/// child in an [Expanded] widget.
///
/// The [Row] widget does not scroll (and in general it is considered an error
/// to have more children in a [Row] than will fit in the available room). If
/// you have a line of widgets and want them to be able to scroll if there is
/// insufficient room, consider using a [ListView].
///
/// For a vertical variant, see [Column].
///
/// If you only have one child, then consider using [Align] or [Center] to
/// position the child.
///
/// By default, [crossAxisAlignment] is [CrossAxisAlignment.center], which
/// centers the children in the vertical axis. If several of the children
/// contain text, this is likely to make them visually misaligned if
/// they have different font metrics (for example because they differ in
/// [TextStyle.fontSize] or other [TextStyle] properties, or because
/// they use different fonts due to being written in different scripts).
/// Consider using [CrossAxisAlignment.baseline] instead.
///
/// {@tool snippet}
///
/// This example divides the available space into three (horizontally), and
/// places text centered in the first two cells and the Flutter logo centered in
/// the third:
///
/// 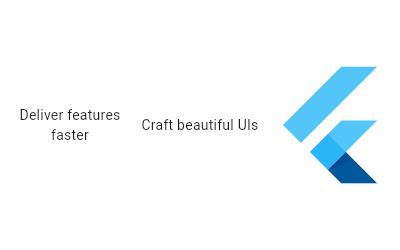
///
/// ```dart
/// const Row(
/// children: <Widget>[
/// Expanded(
/// child: Text('Deliver features faster', textAlign: TextAlign.center),
/// ),
/// Expanded(
/// child: Text('Craft beautiful UIs', textAlign: TextAlign.center),
/// ),
/// Expanded(
/// child: FittedBox(
/// child: FlutterLogo(),
/// ),
/// ),
/// ],
/// )
/// ```
/// {@end-tool}
///
/// ## Troubleshooting
///
/// ### Why does my row have a yellow and black warning stripe?
///
/// If the non-flexible contents of the row (those that are not wrapped in
/// [Expanded] or [Flexible] widgets) are together wider than the row itself,
/// then the row is said to have overflowed. When a row overflows, the row does
/// not have any remaining space to share between its [Expanded] and [Flexible]
/// children. The row reports this by drawing a yellow and black striped
/// warning box on the edge that is overflowing. If there is room on the outside
/// of the row, the amount of overflow is printed in red lettering.
///
/// #### Story time
///
/// Suppose, for instance, that you had this code:
///
/// ```dart
/// const Row(
/// children: <Widget>[
/// FlutterLogo(),
/// Text("Flutter's hot reload helps you quickly and easily experiment, build UIs, add features, and fix bug faster. Experience sub-second reload times, without losing state, on emulators, simulators, and hardware for iOS and Android."),
/// Icon(Icons.sentiment_very_satisfied),
/// ],
/// )
/// ```
///
/// The row first asks its first child, the [FlutterLogo], to lay out, at
/// whatever size the logo would like. The logo is friendly and happily decides
/// to be 24 pixels to a side. This leaves lots of room for the next child. The
/// row then asks that next child, the text, to lay out, at whatever size it
/// thinks is best.
///
/// At this point, the text, not knowing how wide is too wide, says "Ok, I will
/// be thiiiiiiiiiiiiiiiiiiiis wide.", and goes well beyond the space that the
/// row has available, not wrapping. The row responds, "That's not fair, now I
/// have no more room available for my other children!", and gets angry and
/// sprouts a yellow and black strip.
///
/// 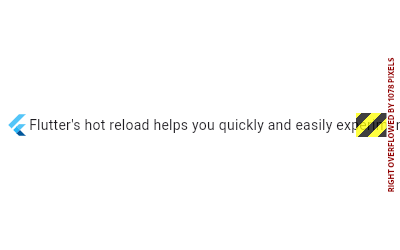
///
/// The fix is to wrap the second child in an [Expanded] widget, which tells the
/// row that the child should be given the remaining room:
///
/// ```dart
/// const Row(
/// children: <Widget>[
/// FlutterLogo(),
/// Expanded(
/// child: Text("Flutter's hot reload helps you quickly and easily experiment, build UIs, add features, and fix bug faster. Experience sub-second reload times, without losing state, on emulators, simulators, and hardware for iOS and Android."),
/// ),
/// Icon(Icons.sentiment_very_satisfied),
/// ],
/// )
/// ```
///
/// Now, the row first asks the logo to lay out, and then asks the _icon_ to lay
/// out. The [Icon], like the logo, is happy to take on a reasonable size (also
/// 24 pixels, not coincidentally, since both [FlutterLogo] and [Icon] honor the
/// ambient [IconTheme]). This leaves some room left over, and now the row tells
/// the text exactly how wide to be: the exact width of the remaining space. The
/// text, now happy to comply to a reasonable request, wraps the text within
/// that width, and you end up with a paragraph split over several lines.
///
/// 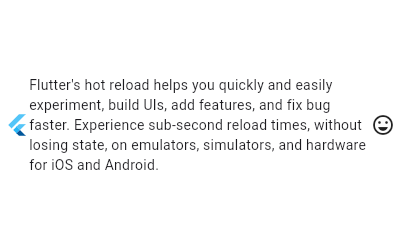
///
/// The [textDirection] property controls the direction that children are rendered in.
/// [TextDirection.ltr] is the default [textDirection] of [Row] children, so the first
/// child is rendered at the `start` of the [Row], to the left, with subsequent children
/// following to the right. If you want to order children in the opposite
/// direction (right to left), then [textDirection] can be set to
/// [TextDirection.rtl]. This is shown in the example below
///
/// ```dart
/// const Row(
/// textDirection: TextDirection.rtl,
/// children: <Widget>[
/// FlutterLogo(),
/// Expanded(
/// child: Text("Flutter's hot reload helps you quickly and easily experiment, build UIs, add features, and fix bug faster. Experience sub-second reload times, without losing state, on emulators, simulators, and hardware for iOS and Android."),
/// ),
/// Icon(Icons.sentiment_very_satisfied),
/// ],
/// )
/// ```
///
/// 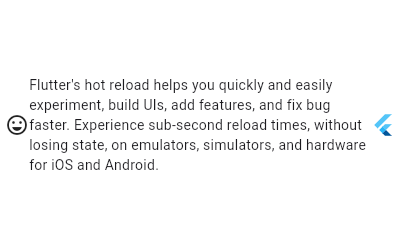
///
/// ## Layout algorithm
///
/// _This section describes how a [Row] is rendered by the framework._
/// _See [BoxConstraints] for an introduction to box layout models._
///
/// Layout for a [Row] proceeds in six steps:
///
/// 1. Layout each child with a null or zero flex factor (e.g., those that are
/// not [Expanded]) with unbounded horizontal constraints and the incoming
/// vertical constraints. If the [crossAxisAlignment] is
/// [CrossAxisAlignment.stretch], instead use tight vertical constraints that
/// match the incoming max height.
/// 2. Divide the remaining horizontal space among the children with non-zero
/// flex factors (e.g., those that are [Expanded]) according to their flex
/// factor. For example, a child with a flex factor of 2.0 will receive twice
/// the amount of horizontal space as a child with a flex factor of 1.0.
/// 3. Layout each of the remaining children with the same vertical constraints
/// as in step 1, but instead of using unbounded horizontal constraints, use
/// horizontal constraints based on the amount of space allocated in step 2.
/// Children with [Flexible.fit] properties that are [FlexFit.tight] are
/// given tight constraints (i.e., forced to fill the allocated space), and
/// children with [Flexible.fit] properties that are [FlexFit.loose] are
/// given loose constraints (i.e., not forced to fill the allocated space).
/// 4. The height of the [Row] is the maximum height of the children (which will
/// always satisfy the incoming vertical constraints).
/// 5. The width of the [Row] is determined by the [mainAxisSize] property. If
/// the [mainAxisSize] property is [MainAxisSize.max], then the width of the
/// [Row] is the max width of the incoming constraints. If the [mainAxisSize]
/// property is [MainAxisSize.min], then the width of the [Row] is the sum
/// of widths of the children (subject to the incoming constraints).
/// 6. Determine the position for each child according to the
/// [mainAxisAlignment] and the [crossAxisAlignment]. For example, if the
/// [mainAxisAlignment] is [MainAxisAlignment.spaceBetween], any horizontal
/// space that has not been allocated to children is divided evenly and
/// placed between the children.
///
/// See also:
///
/// * [Column], for a vertical equivalent.
/// * [Flex], if you don't know in advance if you want a horizontal or vertical
/// arrangement.
/// * [Expanded], to indicate children that should take all the remaining room.
/// * [Flexible], to indicate children that should share the remaining room but
/// that may by sized smaller (leaving some remaining room unused).
/// * [Spacer], a widget that takes up space proportional to its flex value.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class Row extends Flex {
/// Creates a horizontal array of children.
///
/// If [crossAxisAlignment] is [CrossAxisAlignment.baseline], then
/// [textBaseline] must not be null.
///
/// The [textDirection] argument defaults to the ambient [Directionality], if
/// any. If there is no ambient directionality, and a text direction is going
/// to be necessary to determine the layout order (which is always the case
/// unless the row has no children or only one child) or to disambiguate
/// `start` or `end` values for the [mainAxisAlignment], the [textDirection]
/// must not be null.
const Row({
super.key,
super.mainAxisAlignment,
super.mainAxisSize,
super.crossAxisAlignment,
super.textDirection,
super.verticalDirection,
super.textBaseline, // NO DEFAULT: we don't know what the text's baseline should be
super.children,
}) : super(
direction: Axis.horizontal,
);
}
/// A widget that displays its children in a vertical array.
///
/// To cause a child to expand to fill the available vertical space, wrap the
/// child in an [Expanded] widget.
///
/// The [Column] widget does not scroll (and in general it is considered an error
/// to have more children in a [Column] than will fit in the available room). If
/// you have a line of widgets and want them to be able to scroll if there is
/// insufficient room, consider using a [ListView].
///
/// For a horizontal variant, see [Row].
///
/// If you only have one child, then consider using [Align] or [Center] to
/// position the child.
///
/// {@tool snippet}
///
/// This example uses a [Column] to arrange three widgets vertically, the last
/// being made to fill all the remaining space.
///
/// 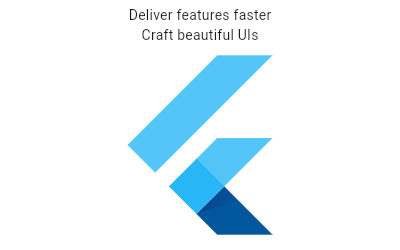
///
/// ```dart
/// const Column(
/// children: <Widget>[
/// Text('Deliver features faster'),
/// Text('Craft beautiful UIs'),
/// Expanded(
/// child: FittedBox(
/// child: FlutterLogo(),
/// ),
/// ),
/// ],
/// )
/// ```
/// {@end-tool}
/// {@tool snippet}
///
/// In the sample above, the text and the logo are centered on each line. In the
/// following example, the [crossAxisAlignment] is set to
/// [CrossAxisAlignment.start], so that the children are left-aligned. The
/// [mainAxisSize] is set to [MainAxisSize.min], so that the column shrinks to
/// fit the children.
///
/// 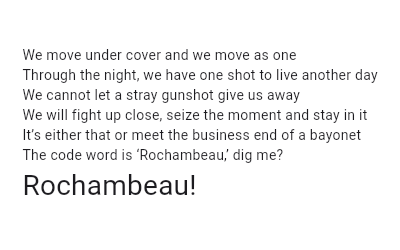
///
/// ```dart
/// Column(
/// crossAxisAlignment: CrossAxisAlignment.start,
/// mainAxisSize: MainAxisSize.min,
/// children: <Widget>[
/// const Text('We move under cover and we move as one'),
/// const Text('Through the night, we have one shot to live another day'),
/// const Text('We cannot let a stray gunshot give us away'),
/// const Text('We will fight up close, seize the moment and stay in it'),
/// const Text("It's either that or meet the business end of a bayonet"),
/// const Text("The code word is 'Rochambeau,' dig me?"),
/// Text('Rochambeau!', style: DefaultTextStyle.of(context).style.apply(fontSizeFactor: 2.0)),
/// ],
/// )
/// ```
/// {@end-tool}
///
/// ## Troubleshooting
///
/// ### When the incoming vertical constraints are unbounded
///
/// When a [Column] has one or more [Expanded] or [Flexible] children, and is
/// placed in another [Column], or in a [ListView], or in some other context
/// that does not provide a maximum height constraint for the [Column], you will
/// get an exception at runtime saying that there are children with non-zero
/// flex but the vertical constraints are unbounded.
///
/// The problem, as described in the details that accompany that exception, is
/// that using [Flexible] or [Expanded] means that the remaining space after
/// laying out all the other children must be shared equally, but if the
/// incoming vertical constraints are unbounded, there is infinite remaining
/// space.
///
/// The key to solving this problem is usually to determine why the [Column] is
/// receiving unbounded vertical constraints.
///
/// One common reason for this to happen is that the [Column] has been placed in
/// another [Column] (without using [Expanded] or [Flexible] around the inner
/// nested [Column]). When a [Column] lays out its non-flex children (those that
/// have neither [Expanded] or [Flexible] around them), it gives them unbounded
/// constraints so that they can determine their own dimensions (passing
/// unbounded constraints usually signals to the child that it should
/// shrink-wrap its contents). The solution in this case is typically to just
/// wrap the inner column in an [Expanded] to indicate that it should take the
/// remaining space of the outer column, rather than being allowed to take any
/// amount of room it desires.
///
/// Another reason for this message to be displayed is nesting a [Column] inside
/// a [ListView] or other vertical scrollable. In that scenario, there really is
/// infinite vertical space (the whole point of a vertical scrolling list is to
/// allow infinite space vertically). In such scenarios, it is usually worth
/// examining why the inner [Column] should have an [Expanded] or [Flexible]
/// child: what size should the inner children really be? The solution in this
/// case is typically to remove the [Expanded] or [Flexible] widgets from around
/// the inner children.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=jckqXR5CrPI}
///
/// For more discussion about constraints, see [BoxConstraints].
///
/// ### The yellow and black striped banner
///
/// When the contents of a [Column] exceed the amount of space available, the
/// [Column] overflows, and the contents are clipped. In debug mode, a yellow
/// and black striped bar is rendered at the overflowing edge to indicate the
/// problem, and a message is printed below the [Column] saying how much
/// overflow was detected.
///
/// The usual solution is to use a [ListView] rather than a [Column], to enable
/// the contents to scroll when vertical space is limited.
///
/// ## Layout algorithm
///
/// _This section describes how a [Column] is rendered by the framework._
/// _See [BoxConstraints] for an introduction to box layout models._
///
/// Layout for a [Column] proceeds in six steps:
///
/// 1. Layout each child with a null or zero flex factor (e.g., those that are
/// not [Expanded]) with unbounded vertical constraints and the incoming
/// horizontal constraints. If the [crossAxisAlignment] is
/// [CrossAxisAlignment.stretch], instead use tight horizontal constraints
/// that match the incoming max width.
/// 2. Divide the remaining vertical space among the children with non-zero
/// flex factors (e.g., those that are [Expanded]) according to their flex
/// factor. For example, a child with a flex factor of 2.0 will receive twice
/// the amount of vertical space as a child with a flex factor of 1.0.
/// 3. Layout each of the remaining children with the same horizontal
/// constraints as in step 1, but instead of using unbounded vertical
/// constraints, use vertical constraints based on the amount of space
/// allocated in step 2. Children with [Flexible.fit] properties that are
/// [FlexFit.tight] are given tight constraints (i.e., forced to fill the
/// allocated space), and children with [Flexible.fit] properties that are
/// [FlexFit.loose] are given loose constraints (i.e., not forced to fill the
/// allocated space).
/// 4. The width of the [Column] is the maximum width of the children (which
/// will always satisfy the incoming horizontal constraints).
/// 5. The height of the [Column] is determined by the [mainAxisSize] property.
/// If the [mainAxisSize] property is [MainAxisSize.max], then the height of
/// the [Column] is the max height of the incoming constraints. If the
/// [mainAxisSize] property is [MainAxisSize.min], then the height of the
/// [Column] is the sum of heights of the children (subject to the incoming
/// constraints).
/// 6. Determine the position for each child according to the
/// [mainAxisAlignment] and the [crossAxisAlignment]. For example, if the
/// [mainAxisAlignment] is [MainAxisAlignment.spaceBetween], any vertical
/// space that has not been allocated to children is divided evenly and
/// placed between the children.
///
/// See also:
///
/// * [Row], for a horizontal equivalent.
/// * [Flex], if you don't know in advance if you want a horizontal or vertical
/// arrangement.
/// * [Expanded], to indicate children that should take all the remaining room.
/// * [Flexible], to indicate children that should share the remaining room but
/// that may size smaller (leaving some remaining room unused).
/// * [SingleChildScrollView], whose documentation discusses some ways to
/// use a [Column] inside a scrolling container.
/// * [Spacer], a widget that takes up space proportional to its flex value.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class Column extends Flex {
/// Creates a vertical array of children.
///
/// If [crossAxisAlignment] is [CrossAxisAlignment.baseline], then
/// [textBaseline] must not be null.
///
/// The [textDirection] argument defaults to the ambient [Directionality], if
/// any. If there is no ambient directionality, and a text direction is going
/// to be necessary to disambiguate `start` or `end` values for the
/// [crossAxisAlignment], the [textDirection] must not be null.
const Column({
super.key,
super.mainAxisAlignment,
super.mainAxisSize,
super.crossAxisAlignment,
super.textDirection,
super.verticalDirection,
super.textBaseline,
super.children,
}) : super(
direction: Axis.vertical,
);
}
/// A widget that controls how a child of a [Row], [Column], or [Flex] flexes.
///
/// Using a [Flexible] widget gives a child of a [Row], [Column], or [Flex]
/// the flexibility to expand to fill the available space in the main axis
/// (e.g., horizontally for a [Row] or vertically for a [Column]), but, unlike
/// [Expanded], [Flexible] does not require the child to fill the available
/// space.
///
/// A [Flexible] widget must be a descendant of a [Row], [Column], or [Flex],
/// and the path from the [Flexible] widget to its enclosing [Row], [Column], or
/// [Flex] must contain only [StatelessWidget]s or [StatefulWidget]s (not other
/// kinds of widgets, like [RenderObjectWidget]s).
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=CI7x0mAZiY0}
///
/// See also:
///
/// * [Expanded], which forces the child to expand to fill the available space.
/// * [Spacer], a widget that takes up space proportional to its flex value.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class Flexible extends ParentDataWidget<FlexParentData> {
/// Creates a widget that controls how a child of a [Row], [Column], or [Flex]
/// flexes.
const Flexible({
super.key,
this.flex = 1,
this.fit = FlexFit.loose,
required super.child,
});
/// The flex factor to use for this child.
///
/// If null or zero, the child is inflexible and determines its own size. If
/// non-zero, the amount of space the child can occupy in the main axis is
/// determined by dividing the free space (after placing the inflexible
/// children) according to the flex factors of the flexible children.
final int flex;
/// How a flexible child is inscribed into the available space.
///
/// If [flex] is non-zero, the [fit] determines whether the child fills the
/// space the parent makes available during layout. If the fit is
/// [FlexFit.tight], the child is required to fill the available space. If the
/// fit is [FlexFit.loose], the child can be at most as large as the available
/// space (but is allowed to be smaller).
final FlexFit fit;
@override
void applyParentData(RenderObject renderObject) {
assert(renderObject.parentData is FlexParentData);
final FlexParentData parentData = renderObject.parentData! as FlexParentData;
bool needsLayout = false;
if (parentData.flex != flex) {
parentData.flex = flex;
needsLayout = true;
}
if (parentData.fit != fit) {
parentData.fit = fit;
needsLayout = true;
}
if (needsLayout) {
final RenderObject? targetParent = renderObject.parent;
if (targetParent is RenderObject) {
targetParent.markNeedsLayout();
}
}
}
@override
Type get debugTypicalAncestorWidgetClass => Flex;
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(IntProperty('flex', flex));
}
}
/// A widget that expands a child of a [Row], [Column], or [Flex]
/// so that the child fills the available space.
///
/// Using an [Expanded] widget makes a child of a [Row], [Column], or [Flex]
/// expand to fill the available space along the main axis (e.g., horizontally for
/// a [Row] or vertically for a [Column]). If multiple children are expanded,
/// the available space is divided among them according to the [flex] factor.
///
/// An [Expanded] widget must be a descendant of a [Row], [Column], or [Flex],
/// and the path from the [Expanded] widget to its enclosing [Row], [Column], or
/// [Flex] must contain only [StatelessWidget]s or [StatefulWidget]s (not other
/// kinds of widgets, like [RenderObjectWidget]s).
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=_rnZaagadyo}
///
/// {@tool dartpad}
/// This example shows how to use an [Expanded] widget in a [Column] so that
/// its middle child, a [Container] here, expands to fill the space.
///
/// 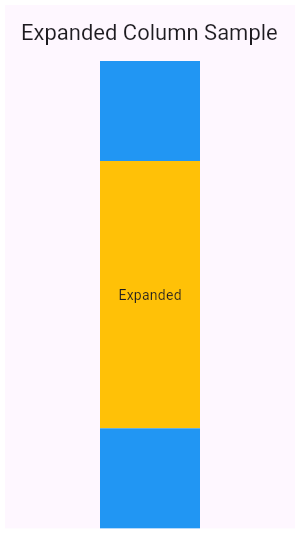
///
/// ** See code in examples/api/lib/widgets/basic/expanded.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// This example shows how to use an [Expanded] widget in a [Row] with multiple
/// children expanded, utilizing the [flex] factor to prioritize available space.
///
/// 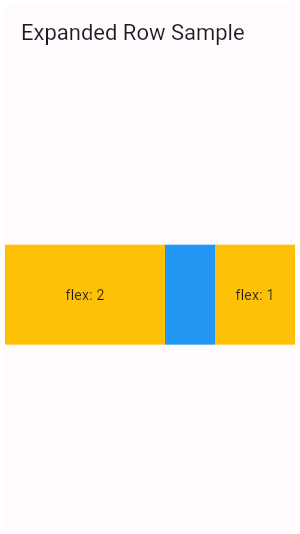
///
/// ** See code in examples/api/lib/widgets/basic/expanded.1.dart **
/// {@end-tool}
///
/// See also:
///
/// * [Flexible], which does not force the child to fill the available space.
/// * [Spacer], a widget that takes up space proportional to its flex value.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class Expanded extends Flexible {
/// Creates a widget that expands a child of a [Row], [Column], or [Flex]
/// so that the child fills the available space along the flex widget's
/// main axis.
const Expanded({
super.key,
super.flex,
required super.child,
}) : super(fit: FlexFit.tight);
}
/// A widget that displays its children in multiple horizontal or vertical runs.
///
/// A [Wrap] lays out each child and attempts to place the child adjacent to the
/// previous child in the main axis, given by [direction], leaving [spacing]
/// space in between. If there is not enough space to fit the child, [Wrap]
/// creates a new _run_ adjacent to the existing children in the cross axis.
///
/// After all the children have been allocated to runs, the children within the
/// runs are positioned according to the [alignment] in the main axis and
/// according to the [crossAxisAlignment] in the cross axis.
///
/// The runs themselves are then positioned in the cross axis according to the
/// [runSpacing] and [runAlignment].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=z5iw2SeFx2M}
///
/// {@tool snippet}
///
/// This example renders some [Chip]s representing four contacts in a [Wrap] so
/// that they flow across lines as necessary.
///
/// ```dart
/// Wrap(
/// spacing: 8.0, // gap between adjacent chips
/// runSpacing: 4.0, // gap between lines
/// children: <Widget>[
/// Chip(
/// avatar: CircleAvatar(backgroundColor: Colors.blue.shade900, child: const Text('AH')),
/// label: const Text('Hamilton'),
/// ),
/// Chip(
/// avatar: CircleAvatar(backgroundColor: Colors.blue.shade900, child: const Text('ML')),
/// label: const Text('Lafayette'),
/// ),
/// Chip(
/// avatar: CircleAvatar(backgroundColor: Colors.blue.shade900, child: const Text('HM')),
/// label: const Text('Mulligan'),
/// ),
/// Chip(
/// avatar: CircleAvatar(backgroundColor: Colors.blue.shade900, child: const Text('JL')),
/// label: const Text('Laurens'),
/// ),
/// ],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [Row], which places children in one line, and gives control over their
/// alignment and spacing.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class Wrap extends MultiChildRenderObjectWidget {
/// Creates a wrap layout.
///
/// By default, the wrap layout is horizontal and both the children and the
/// runs are aligned to the start.
///
/// The [textDirection] argument defaults to the ambient [Directionality], if
/// any. If there is no ambient directionality, and a text direction is going
/// to be necessary to decide which direction to lay the children in or to
/// disambiguate `start` or `end` values for the main or cross axis
/// directions, the [textDirection] must not be null.
const Wrap({
super.key,
this.direction = Axis.horizontal,
this.alignment = WrapAlignment.start,
this.spacing = 0.0,
this.runAlignment = WrapAlignment.start,
this.runSpacing = 0.0,
this.crossAxisAlignment = WrapCrossAlignment.start,
this.textDirection,
this.verticalDirection = VerticalDirection.down,
this.clipBehavior = Clip.none,
super.children,
});
/// The direction to use as the main axis.
///
/// For example, if [direction] is [Axis.horizontal], the default, the
/// children are placed adjacent to one another in a horizontal run until the
/// available horizontal space is consumed, at which point a subsequent
/// children are placed in a new run vertically adjacent to the previous run.
final Axis direction;
/// How the children within a run should be placed in the main axis.
///
/// For example, if [alignment] is [WrapAlignment.center], the children in
/// each run are grouped together in the center of their run in the main axis.
///
/// Defaults to [WrapAlignment.start].
///
/// See also:
///
/// * [runAlignment], which controls how the runs are placed relative to each
/// other in the cross axis.
/// * [crossAxisAlignment], which controls how the children within each run
/// are placed relative to each other in the cross axis.
final WrapAlignment alignment;
/// How much space to place between children in a run in the main axis.
///
/// For example, if [spacing] is 10.0, the children will be spaced at least
/// 10.0 logical pixels apart in the main axis.
///
/// If there is additional free space in a run (e.g., because the wrap has a
/// minimum size that is not filled or because some runs are longer than
/// others), the additional free space will be allocated according to the
/// [alignment].
///
/// Defaults to 0.0.
final double spacing;
/// How the runs themselves should be placed in the cross axis.
///
/// For example, if [runAlignment] is [WrapAlignment.center], the runs are
/// grouped together in the center of the overall [Wrap] in the cross axis.
///
/// Defaults to [WrapAlignment.start].
///
/// See also:
///
/// * [alignment], which controls how the children within each run are placed
/// relative to each other in the main axis.
/// * [crossAxisAlignment], which controls how the children within each run
/// are placed relative to each other in the cross axis.
final WrapAlignment runAlignment;
/// How much space to place between the runs themselves in the cross axis.
///
/// For example, if [runSpacing] is 10.0, the runs will be spaced at least
/// 10.0 logical pixels apart in the cross axis.
///
/// If there is additional free space in the overall [Wrap] (e.g., because
/// the wrap has a minimum size that is not filled), the additional free space
/// will be allocated according to the [runAlignment].
///
/// Defaults to 0.0.
final double runSpacing;
/// How the children within a run should be aligned relative to each other in
/// the cross axis.
///
/// For example, if this is set to [WrapCrossAlignment.end], and the
/// [direction] is [Axis.horizontal], then the children within each
/// run will have their bottom edges aligned to the bottom edge of the run.
///
/// Defaults to [WrapCrossAlignment.start].
///
/// See also:
///
/// * [alignment], which controls how the children within each run are placed
/// relative to each other in the main axis.
/// * [runAlignment], which controls how the runs are placed relative to each
/// other in the cross axis.
final WrapCrossAlignment crossAxisAlignment;
/// Determines the order to lay children out horizontally and how to interpret
/// `start` and `end` in the horizontal direction.
///
/// Defaults to the ambient [Directionality].
///
/// If the [direction] is [Axis.horizontal], this controls order in which the
/// children are positioned (left-to-right or right-to-left), and the meaning
/// of the [alignment] property's [WrapAlignment.start] and
/// [WrapAlignment.end] values.
///
/// If the [direction] is [Axis.horizontal], and either the
/// [alignment] is either [WrapAlignment.start] or [WrapAlignment.end], or
/// there's more than one child, then the [textDirection] (or the ambient
/// [Directionality]) must not be null.
///
/// If the [direction] is [Axis.vertical], this controls the order in which
/// runs are positioned, the meaning of the [runAlignment] property's
/// [WrapAlignment.start] and [WrapAlignment.end] values, as well as the
/// [crossAxisAlignment] property's [WrapCrossAlignment.start] and
/// [WrapCrossAlignment.end] values.
///
/// If the [direction] is [Axis.vertical], and either the
/// [runAlignment] is either [WrapAlignment.start] or [WrapAlignment.end], the
/// [crossAxisAlignment] is either [WrapCrossAlignment.start] or
/// [WrapCrossAlignment.end], or there's more than one child, then the
/// [textDirection] (or the ambient [Directionality]) must not be null.
final TextDirection? textDirection;
/// Determines the order to lay children out vertically and how to interpret
/// `start` and `end` in the vertical direction.
///
/// If the [direction] is [Axis.vertical], this controls which order children
/// are painted in (down or up), the meaning of the [alignment] property's
/// [WrapAlignment.start] and [WrapAlignment.end] values.
///
/// If the [direction] is [Axis.vertical], and either the [alignment]
/// is either [WrapAlignment.start] or [WrapAlignment.end], or there's
/// more than one child, then the [verticalDirection] must not be null.
///
/// If the [direction] is [Axis.horizontal], this controls the order in which
/// runs are positioned, the meaning of the [runAlignment] property's
/// [WrapAlignment.start] and [WrapAlignment.end] values, as well as the
/// [crossAxisAlignment] property's [WrapCrossAlignment.start] and
/// [WrapCrossAlignment.end] values.
///
/// If the [direction] is [Axis.horizontal], and either the
/// [runAlignment] is either [WrapAlignment.start] or [WrapAlignment.end], the
/// [crossAxisAlignment] is either [WrapCrossAlignment.start] or
/// [WrapCrossAlignment.end], or there's more than one child, then the
/// [verticalDirection] must not be null.
final VerticalDirection verticalDirection;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.none].
final Clip clipBehavior;
@override
RenderWrap createRenderObject(BuildContext context) {
return RenderWrap(
direction: direction,
alignment: alignment,
spacing: spacing,
runAlignment: runAlignment,
runSpacing: runSpacing,
crossAxisAlignment: crossAxisAlignment,
textDirection: textDirection ?? Directionality.maybeOf(context),
verticalDirection: verticalDirection,
clipBehavior: clipBehavior,
);
}
@override
void updateRenderObject(BuildContext context, RenderWrap renderObject) {
renderObject
..direction = direction
..alignment = alignment
..spacing = spacing
..runAlignment = runAlignment
..runSpacing = runSpacing
..crossAxisAlignment = crossAxisAlignment
..textDirection = textDirection ?? Directionality.maybeOf(context)
..verticalDirection = verticalDirection
..clipBehavior = clipBehavior;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<Axis>('direction', direction));
properties.add(EnumProperty<WrapAlignment>('alignment', alignment));
properties.add(DoubleProperty('spacing', spacing));
properties.add(EnumProperty<WrapAlignment>('runAlignment', runAlignment));
properties.add(DoubleProperty('runSpacing', runSpacing));
properties.add(EnumProperty<WrapCrossAlignment>('crossAxisAlignment', crossAxisAlignment));
properties.add(EnumProperty<TextDirection>('textDirection', textDirection, defaultValue: null));
properties.add(EnumProperty<VerticalDirection>('verticalDirection', verticalDirection, defaultValue: VerticalDirection.down));
}
}
/// A widget that sizes and positions children efficiently, according to the
/// logic in a [FlowDelegate].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=NG6pvXpnIso}
///
/// Flow layouts are optimized for repositioning children using transformation
/// matrices.
///
/// The flow container is sized independently from the children by the
/// [FlowDelegate.getSize] function of the delegate. The children are then sized
/// independently given the constraints from the
/// [FlowDelegate.getConstraintsForChild] function.
///
/// Rather than positioning the children during layout, the children are
/// positioned using transformation matrices during the paint phase using the
/// matrices from the [FlowDelegate.paintChildren] function. The children can be
/// repositioned efficiently by only _repainting_ the flow, which happens
/// without the children being laid out again (contrast this with a [Stack],
/// which does the sizing and positioning together during layout).
///
/// The most efficient way to trigger a repaint of the flow is to supply an
/// animation to the constructor of the [FlowDelegate]. The flow will listen to
/// this animation and repaint whenever the animation ticks, avoiding both the
/// build and layout phases of the pipeline.
///
/// {@tool dartpad}
/// This example uses the [Flow] widget to create a menu that opens and closes
/// as it is interacted with, shown above. The color of the button in the menu
/// changes to indicate which one has been selected.
///
/// ** See code in examples/api/lib/widgets/basic/flow.0.dart **
/// {@end-tool}
///
/// ## Hit testing and hidden [Flow] widgets
///
/// The [Flow] widget recomputes its children's positions (as used by hit
/// testing) during the _paint_ phase rather than during the _layout_ phase.
///
/// Widgets like [Opacity] avoid painting their children when those children
/// would be invisible due to their opacity being zero.
///
/// Unfortunately, this means that hiding a [Flow] widget using an [Opacity]
/// widget will cause bugs when the user attempts to interact with the hidden
/// region, for example, by tapping it or clicking it.
///
/// Such bugs will manifest either as out-of-date geometry (taps going to
/// different widgets than might be expected by the currently-specified
/// [FlowDelegate]s), or exceptions (e.g. if the last time the [Flow] was
/// painted, a different set of children was specified).
///
/// To avoid this, when hiding a [Flow] widget with an [Opacity] widget (or
/// [AnimatedOpacity] or similar), it is wise to also disable hit testing on the
/// widget by using [IgnorePointer]. This is generally good advice anyway as
/// hit-testing invisible widgets is often confusing for the user.
///
/// See also:
///
/// * [Wrap], which provides the layout model that some other frameworks call
/// "flow", and is otherwise unrelated to [Flow].
/// * [Stack], which arranges children relative to the edges of the container.
/// * [CustomSingleChildLayout], which uses a delegate to control the layout of
/// a single child.
/// * [CustomMultiChildLayout], which uses a delegate to position multiple
/// children.
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class Flow extends MultiChildRenderObjectWidget {
/// Creates a flow layout.
///
/// Wraps each of the given children in a [RepaintBoundary] to avoid
/// repainting the children when the flow repaints.
Flow({
super.key,
required this.delegate,
List<Widget> children = const <Widget>[],
this.clipBehavior = Clip.hardEdge,
}) : super(children: RepaintBoundary.wrapAll(children));
// https://github.com/dart-lang/sdk/issues/29277
/// Creates a flow layout.
///
/// Does not wrap the given children in repaint boundaries, unlike the default
/// constructor. Useful when the child is trivial to paint or already contains
/// a repaint boundary.
const Flow.unwrapped({
super.key,
required this.delegate,
super.children,
this.clipBehavior = Clip.hardEdge,
});
/// The delegate that controls the transformation matrices of the children.
final FlowDelegate delegate;
/// {@macro flutter.material.Material.clipBehavior}
///
/// Defaults to [Clip.none].
final Clip clipBehavior;
@override
RenderFlow createRenderObject(BuildContext context) => RenderFlow(delegate: delegate, clipBehavior: clipBehavior);
@override
void updateRenderObject(BuildContext context, RenderFlow renderObject) {
renderObject.delegate = delegate;
renderObject.clipBehavior = clipBehavior;
}
}
/// A paragraph of rich text.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=rykDVh-QFfw}
///
/// The [RichText] widget displays text that uses multiple different styles. The
/// text to display is described using a tree of [TextSpan] objects, each of
/// which has an associated style that is used for that subtree. The text might
/// break across multiple lines or might all be displayed on the same line
/// depending on the layout constraints.
///
/// Text displayed in a [RichText] widget must be explicitly styled. When
/// picking which style to use, consider using [DefaultTextStyle.of] the current
/// [BuildContext] to provide defaults. For more details on how to style text in
/// a [RichText] widget, see the documentation for [TextStyle].
///
/// Consider using the [Text] widget to integrate with the [DefaultTextStyle]
/// automatically. When all the text uses the same style, the default constructor
/// is less verbose. The [Text.rich] constructor allows you to style multiple
/// spans with the default text style while still allowing specified styles per
/// span.
///
/// {@tool snippet}
///
/// This sample demonstrates how to mix and match text with different text
/// styles using the [RichText] Widget. It displays the text "Hello bold world,"
/// emphasizing the word "bold" using a bold font weight.
///
/// 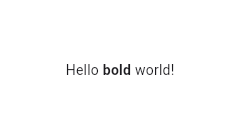
///
/// ```dart
/// RichText(
/// text: TextSpan(
/// text: 'Hello ',
/// style: DefaultTextStyle.of(context).style,
/// children: const <TextSpan>[
/// TextSpan(text: 'bold', style: TextStyle(fontWeight: FontWeight.bold)),
/// TextSpan(text: ' world!'),
/// ],
/// ),
/// )
/// ```
/// {@end-tool}
///
/// ## Selections
///
/// To make this [RichText] Selectable, the [RichText] needs to be in the
/// subtree of a [SelectionArea] or [SelectableRegion] and a
/// [SelectionRegistrar] needs to be assigned to the
/// [RichText.selectionRegistrar]. One can use
/// [SelectionContainer.maybeOf] to get the [SelectionRegistrar] from a
/// context. This enables users to select the text in [RichText]s with mice or
/// touch events.
///
/// The [selectionColor] also needs to be set if the selection is enabled to
/// draw the selection highlights.
///
/// {@tool snippet}
///
/// This sample demonstrates how to assign a [SelectionRegistrar] for RichTexts
/// in the SelectionArea subtree.
///
/// 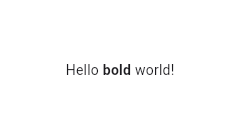
///
/// ```dart
/// RichText(
/// text: const TextSpan(text: 'Hello'),
/// selectionRegistrar: SelectionContainer.maybeOf(context),
/// selectionColor: const Color(0xAF6694e8),
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [TextStyle], which discusses how to style text.
/// * [TextSpan], which is used to describe the text in a paragraph.
/// * [Text], which automatically applies the ambient styles described by a
/// [DefaultTextStyle] to a single string.
/// * [Text.rich], a const text widget that provides similar functionality
/// as [RichText]. [Text.rich] will inherit [TextStyle] from [DefaultTextStyle].
/// * [SelectableRegion], which provides an overview of the selection system.
class RichText extends MultiChildRenderObjectWidget {
/// Creates a paragraph of rich text.
///
/// The [maxLines] property may be null (and indeed defaults to null), but if
/// it is not null, it must be greater than zero.
///
/// The [textDirection], if null, defaults to the ambient [Directionality],
/// which in that case must not be null.
RichText({
super.key,
required this.text,
this.textAlign = TextAlign.start,
this.textDirection,
this.softWrap = true,
this.overflow = TextOverflow.clip,
@Deprecated(
'Use textScaler instead. '
'Use of textScaleFactor was deprecated in preparation for the upcoming nonlinear text scaling support. '
'This feature was deprecated after v3.12.0-2.0.pre.',
)
double textScaleFactor = 1.0,
TextScaler textScaler = TextScaler.noScaling,
this.maxLines,
this.locale,
this.strutStyle,
this.textWidthBasis = TextWidthBasis.parent,
this.textHeightBehavior,
this.selectionRegistrar,
this.selectionColor,
}) : assert(maxLines == null || maxLines > 0),
assert(selectionRegistrar == null || selectionColor != null),
assert(textScaleFactor == 1.0 || identical(textScaler, TextScaler.noScaling), 'Use textScaler instead.'),
textScaler = _effectiveTextScalerFrom(textScaler, textScaleFactor),
super(children: WidgetSpan.extractFromInlineSpan(text, _effectiveTextScalerFrom(textScaler, textScaleFactor)));
static TextScaler _effectiveTextScalerFrom(TextScaler textScaler, double textScaleFactor) {
return switch ((textScaler, textScaleFactor)) {
(final TextScaler scaler, 1.0) => scaler,
(TextScaler.noScaling, final double textScaleFactor) => TextScaler.linear(textScaleFactor),
(final TextScaler scaler, _) => scaler,
};
}
/// The text to display in this widget.
final InlineSpan text;
/// How the text should be aligned horizontally.
final TextAlign textAlign;
/// The directionality of the text.
///
/// This decides how [textAlign] values like [TextAlign.start] and
/// [TextAlign.end] are interpreted.
///
/// This is also used to disambiguate how to render bidirectional text. For
/// example, if the [text] is an English phrase followed by a Hebrew phrase,
/// in a [TextDirection.ltr] context the English phrase will be on the left
/// and the Hebrew phrase to its right, while in a [TextDirection.rtl]
/// context, the English phrase will be on the right and the Hebrew phrase on
/// its left.
///
/// Defaults to the ambient [Directionality], if any. If there is no ambient
/// [Directionality], then this must not be null.
final TextDirection? textDirection;
/// Whether the text should break at soft line breaks.
///
/// If false, the glyphs in the text will be positioned as if there was unlimited horizontal space.
final bool softWrap;
/// How visual overflow should be handled.
final TextOverflow overflow;
/// Deprecated. Will be removed in a future version of Flutter. Use
/// [textScaler] instead.
///
/// The number of font pixels for each logical pixel.
///
/// For example, if the text scale factor is 1.5, text will be 50% larger than
/// the specified font size.
@Deprecated(
'Use textScaler instead. '
'Use of textScaleFactor was deprecated in preparation for the upcoming nonlinear text scaling support. '
'This feature was deprecated after v3.12.0-2.0.pre.',
)
double get textScaleFactor => textScaler.textScaleFactor;
/// {@macro flutter.painting.textPainter.textScaler}
final TextScaler textScaler;
/// An optional maximum number of lines for the text to span, wrapping if necessary.
/// If the text exceeds the given number of lines, it will be truncated according
/// to [overflow].
///
/// If this is 1, text will not wrap. Otherwise, text will be wrapped at the
/// edge of the box.
final int? maxLines;
/// Used to select a font when the same Unicode character can
/// be rendered differently, depending on the locale.
///
/// It's rarely necessary to set this property. By default its value
/// is inherited from the enclosing app with `Localizations.localeOf(context)`.
///
/// See [RenderParagraph.locale] for more information.
final Locale? locale;
/// {@macro flutter.painting.textPainter.strutStyle}
final StrutStyle? strutStyle;
/// {@macro flutter.painting.textPainter.textWidthBasis}
final TextWidthBasis textWidthBasis;
/// {@macro dart.ui.textHeightBehavior}
final ui.TextHeightBehavior? textHeightBehavior;
/// The [SelectionRegistrar] this rich text is subscribed to.
///
/// If this is set, [selectionColor] must be non-null.
final SelectionRegistrar? selectionRegistrar;
/// The color to use when painting the selection.
///
/// This is ignored if [selectionRegistrar] is null.
///
/// See the section on selections in the [RichText] top-level API
/// documentation for more details on enabling selection in [RichText]
/// widgets.
final Color? selectionColor;
@override
RenderParagraph createRenderObject(BuildContext context) {
assert(textDirection != null || debugCheckHasDirectionality(context));
return RenderParagraph(text,
textAlign: textAlign,
textDirection: textDirection ?? Directionality.of(context),
softWrap: softWrap,
overflow: overflow,
textScaler: textScaler,
maxLines: maxLines,
strutStyle: strutStyle,
textWidthBasis: textWidthBasis,
textHeightBehavior: textHeightBehavior,
locale: locale ?? Localizations.maybeLocaleOf(context),
registrar: selectionRegistrar,
selectionColor: selectionColor,
);
}
@override
void updateRenderObject(BuildContext context, RenderParagraph renderObject) {
assert(textDirection != null || debugCheckHasDirectionality(context));
renderObject
..text = text
..textAlign = textAlign
..textDirection = textDirection ?? Directionality.of(context)
..softWrap = softWrap
..overflow = overflow
..textScaler = textScaler
..maxLines = maxLines
..strutStyle = strutStyle
..textWidthBasis = textWidthBasis
..textHeightBehavior = textHeightBehavior
..locale = locale ?? Localizations.maybeLocaleOf(context)
..registrar = selectionRegistrar
..selectionColor = selectionColor;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<TextAlign>('textAlign', textAlign, defaultValue: TextAlign.start));
properties.add(EnumProperty<TextDirection>('textDirection', textDirection, defaultValue: null));
properties.add(FlagProperty('softWrap', value: softWrap, ifTrue: 'wrapping at box width', ifFalse: 'no wrapping except at line break characters', showName: true));
properties.add(EnumProperty<TextOverflow>('overflow', overflow, defaultValue: TextOverflow.clip));
properties.add(DiagnosticsProperty<TextScaler>('textScaler', textScaler, defaultValue: TextScaler.noScaling));
properties.add(IntProperty('maxLines', maxLines, ifNull: 'unlimited'));
properties.add(EnumProperty<TextWidthBasis>('textWidthBasis', textWidthBasis, defaultValue: TextWidthBasis.parent));
properties.add(StringProperty('text', text.toPlainText()));
properties.add(DiagnosticsProperty<Locale>('locale', locale, defaultValue: null));
properties.add(DiagnosticsProperty<StrutStyle>('strutStyle', strutStyle, defaultValue: null));
properties.add(DiagnosticsProperty<TextHeightBehavior>('textHeightBehavior', textHeightBehavior, defaultValue: null));
}
}
/// A widget that displays a [dart:ui.Image] directly.
///
/// The image is painted using [paintImage], which describes the meanings of the
/// various fields on this class in more detail.
///
/// The [image] is not disposed of by this widget. Creators of the widget are
/// expected to call [Image.dispose] on the [image] once the [RawImage] is no
/// longer buildable.
///
/// This widget is rarely used directly. Instead, consider using [Image].
class RawImage extends LeafRenderObjectWidget {
/// Creates a widget that displays an image.
///
/// The [scale], [alignment], [repeat], [matchTextDirection] and [filterQuality] arguments must
/// not be null.
const RawImage({
super.key,
this.image,
this.debugImageLabel,
this.width,
this.height,
this.scale = 1.0,
this.color,
this.opacity,
this.colorBlendMode,
this.fit,
this.alignment = Alignment.center,
this.repeat = ImageRepeat.noRepeat,
this.centerSlice,
this.matchTextDirection = false,
this.invertColors = false,
this.filterQuality = FilterQuality.low,
this.isAntiAlias = false,
});
/// The image to display.
///
/// Since a [RawImage] is stateless, it does not ever dispose this image.
/// Creators of a [RawImage] are expected to call [Image.dispose] on this
/// image handle when the [RawImage] will no longer be needed.
final ui.Image? image;
/// A string identifying the source of the image.
final String? debugImageLabel;
/// If non-null, require the image to have this width.
///
/// If null, the image will pick a size that best preserves its intrinsic
/// aspect ratio.
final double? width;
/// If non-null, require the image to have this height.
///
/// If null, the image will pick a size that best preserves its intrinsic
/// aspect ratio.
final double? height;
/// Specifies the image's scale.
///
/// Used when determining the best display size for the image.
final double scale;
/// If non-null, this color is blended with each image pixel using [colorBlendMode].
final Color? color;
/// If non-null, the value from the [Animation] is multiplied with the opacity
/// of each image pixel before painting onto the canvas.
///
/// This is more efficient than using [FadeTransition] to change the opacity
/// of an image.
final Animation<double>? opacity;
/// Used to set the filterQuality of the image.
///
/// Defaults to [FilterQuality.low] to scale the image, which corresponds to
/// bilinear interpolation.
final FilterQuality filterQuality;
/// Used to combine [color] with this image.
///
/// The default is [BlendMode.srcIn]. In terms of the blend mode, [color] is
/// the source and this image is the destination.
///
/// See also:
///
/// * [BlendMode], which includes an illustration of the effect of each blend mode.
final BlendMode? colorBlendMode;
/// How to inscribe the image into the space allocated during layout.
///
/// The default varies based on the other fields. See the discussion at
/// [paintImage].
final BoxFit? fit;
/// How to align the image within its bounds.
///
/// The alignment aligns the given position in the image to the given position
/// in the layout bounds. For example, an [Alignment] alignment of (-1.0,
/// -1.0) aligns the image to the top-left corner of its layout bounds, while a
/// [Alignment] alignment of (1.0, 1.0) aligns the bottom right of the
/// image with the bottom right corner of its layout bounds. Similarly, an
/// alignment of (0.0, 1.0) aligns the bottom middle of the image with the
/// middle of the bottom edge of its layout bounds.
///
/// To display a subpart of an image, consider using a [CustomPainter] and
/// [Canvas.drawImageRect].
///
/// If the [alignment] is [TextDirection]-dependent (i.e. if it is a
/// [AlignmentDirectional]), then an ambient [Directionality] widget
/// must be in scope.
///
/// Defaults to [Alignment.center].
///
/// See also:
///
/// * [Alignment], a class with convenient constants typically used to
/// specify an [AlignmentGeometry].
/// * [AlignmentDirectional], like [Alignment] for specifying alignments
/// relative to text direction.
final AlignmentGeometry alignment;
/// How to paint any portions of the layout bounds not covered by the image.
final ImageRepeat repeat;
/// The center slice for a nine-patch image.
///
/// The region of the image inside the center slice will be stretched both
/// horizontally and vertically to fit the image into its destination. The
/// region of the image above and below the center slice will be stretched
/// only horizontally and the region of the image to the left and right of
/// the center slice will be stretched only vertically.
final Rect? centerSlice;
/// Whether to paint the image in the direction of the [TextDirection].
///
/// If this is true, then in [TextDirection.ltr] contexts, the image will be
/// drawn with its origin in the top left (the "normal" painting direction for
/// images); and in [TextDirection.rtl] contexts, the image will be drawn with
/// a scaling factor of -1 in the horizontal direction so that the origin is
/// in the top right.
///
/// This is occasionally used with images in right-to-left environments, for
/// images that were designed for left-to-right locales. Be careful, when
/// using this, to not flip images with integral shadows, text, or other
/// effects that will look incorrect when flipped.
///
/// If this is true, there must be an ambient [Directionality] widget in
/// scope.
final bool matchTextDirection;
/// Whether the colors of the image are inverted when drawn.
///
/// Inverting the colors of an image applies a new color filter to the paint.
/// If there is another specified color filter, the invert will be applied
/// after it. This is primarily used for implementing smart invert on iOS.
///
/// See also:
///
/// * [Paint.invertColors], for the dart:ui implementation.
final bool invertColors;
/// Whether to paint the image with anti-aliasing.
///
/// Anti-aliasing alleviates the sawtooth artifact when the image is rotated.
final bool isAntiAlias;
@override
RenderImage createRenderObject(BuildContext context) {
assert((!matchTextDirection && alignment is Alignment) || debugCheckHasDirectionality(context));
assert(
image?.debugGetOpenHandleStackTraces()?.isNotEmpty ?? true,
'Creator of a RawImage disposed of the image when the RawImage still '
'needed it.',
);
return RenderImage(
image: image?.clone(),
debugImageLabel: debugImageLabel,
width: width,
height: height,
scale: scale,
color: color,
opacity: opacity,
colorBlendMode: colorBlendMode,
fit: fit,
alignment: alignment,
repeat: repeat,
centerSlice: centerSlice,
matchTextDirection: matchTextDirection,
textDirection: matchTextDirection || alignment is! Alignment ? Directionality.of(context) : null,
invertColors: invertColors,
isAntiAlias: isAntiAlias,
filterQuality: filterQuality,
);
}
@override
void updateRenderObject(BuildContext context, RenderImage renderObject) {
assert(
image?.debugGetOpenHandleStackTraces()?.isNotEmpty ?? true,
'Creator of a RawImage disposed of the image when the RawImage still '
'needed it.',
);
renderObject
..image = image?.clone()
..debugImageLabel = debugImageLabel
..width = width
..height = height
..scale = scale
..color = color
..opacity = opacity
..colorBlendMode = colorBlendMode
..fit = fit
..alignment = alignment
..repeat = repeat
..centerSlice = centerSlice
..matchTextDirection = matchTextDirection
..textDirection = matchTextDirection || alignment is! Alignment ? Directionality.of(context) : null
..invertColors = invertColors
..isAntiAlias = isAntiAlias
..filterQuality = filterQuality;
}
@override
void didUnmountRenderObject(RenderImage renderObject) {
// Have the render object dispose its image handle.
renderObject.image = null;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<ui.Image>('image', image));
properties.add(DoubleProperty('width', width, defaultValue: null));
properties.add(DoubleProperty('height', height, defaultValue: null));
properties.add(DoubleProperty('scale', scale, defaultValue: 1.0));
properties.add(ColorProperty('color', color, defaultValue: null));
properties.add(DiagnosticsProperty<Animation<double>?>('opacity', opacity, defaultValue: null));
properties.add(EnumProperty<BlendMode>('colorBlendMode', colorBlendMode, defaultValue: null));
properties.add(EnumProperty<BoxFit>('fit', fit, defaultValue: null));
properties.add(DiagnosticsProperty<AlignmentGeometry>('alignment', alignment, defaultValue: null));
properties.add(EnumProperty<ImageRepeat>('repeat', repeat, defaultValue: ImageRepeat.noRepeat));
properties.add(DiagnosticsProperty<Rect>('centerSlice', centerSlice, defaultValue: null));
properties.add(FlagProperty('matchTextDirection', value: matchTextDirection, ifTrue: 'match text direction'));
properties.add(DiagnosticsProperty<bool>('invertColors', invertColors));
properties.add(EnumProperty<FilterQuality>('filterQuality', filterQuality));
}
}
/// A widget that determines the default asset bundle for its descendants.
///
/// For example, used by [Image] to determine which bundle to use for
/// [AssetImage]s if no bundle is specified explicitly.
///
/// {@tool snippet}
///
/// This can be used in tests to override what the current asset bundle is, thus
/// allowing specific resources to be injected into the widget under test.
///
/// For example, a test could create a test asset bundle like this:
///
/// ```dart
/// class TestAssetBundle extends CachingAssetBundle {
/// @override
/// Future<ByteData> load(String key) async {
/// if (key == 'resources/test') {
/// return ByteData.sublistView(utf8.encode('Hello World!'));
/// }
/// return ByteData(0);
/// }
/// }
/// ```
/// {@end-tool}
/// {@tool snippet}
///
/// ...then wrap the widget under test with a [DefaultAssetBundle] using this
/// bundle implementation:
///
/// ```dart
/// // continuing from previous example...
/// await tester.pumpWidget(
/// MaterialApp(
/// home: DefaultAssetBundle(
/// bundle: TestAssetBundle(),
/// child: const TestWidget(),
/// ),
/// ),
/// );
/// ```
/// {@end-tool}
///
/// Assuming that `TestWidget` uses [DefaultAssetBundle.of] to obtain its
/// [AssetBundle], it will now see the `TestAssetBundle`'s "Hello World!" data
/// when requesting the "resources/test" asset.
///
/// See also:
///
/// * [AssetBundle], the interface for asset bundles.
/// * [rootBundle], the default asset bundle.
class DefaultAssetBundle extends InheritedWidget {
/// Creates a widget that determines the default asset bundle for its descendants.
const DefaultAssetBundle({
super.key,
required this.bundle,
required super.child,
});
/// The bundle to use as a default.
final AssetBundle bundle;
/// The bundle from the closest instance of this class that encloses
/// the given context.
///
/// If there is no [DefaultAssetBundle] ancestor widget in the tree
/// at the given context, then this will return the [rootBundle].
///
/// Typical usage is as follows:
///
/// ```dart
/// AssetBundle bundle = DefaultAssetBundle.of(context);
/// ```
static AssetBundle of(BuildContext context) {
final DefaultAssetBundle? result = context.dependOnInheritedWidgetOfExactType<DefaultAssetBundle>();
return result?.bundle ?? rootBundle;
}
@override
bool updateShouldNotify(DefaultAssetBundle oldWidget) => bundle != oldWidget.bundle;
}
/// An adapter for placing a specific [RenderBox] in the widget tree.
///
/// A given render object can be placed at most once in the widget tree. This
/// widget enforces that restriction by keying itself using a [GlobalObjectKey]
/// for the given render object.
///
/// This widget will call [RenderObject.dispose] on the [renderBox] when it is
/// unmounted. After that point, the [renderBox] will be unusable. If any
/// children have been added to the [renderBox], they must be disposed in the
/// [onUnmount] callback.
class WidgetToRenderBoxAdapter extends LeafRenderObjectWidget {
/// Creates an adapter for placing a specific [RenderBox] in the widget tree.
WidgetToRenderBoxAdapter({
required this.renderBox,
this.onBuild,
this.onUnmount,
}) : super(key: GlobalObjectKey(renderBox));
/// The render box to place in the widget tree.
///
/// This widget takes ownership of the render object. When it is unmounted,
/// it also calls [RenderObject.dispose].
final RenderBox renderBox;
/// Called when it is safe to update the render box and its descendants. If
/// you update the RenderObject subtree under this widget outside of
/// invocations of this callback, features like hit-testing will fail as the
/// tree will be dirty.
final VoidCallback? onBuild;
/// Called when it is safe to dispose of children that were manually added to
/// the [renderBox].
///
/// Do not dispose the [renderBox] itself, as it will be disposed by the
/// framework automatically. However, during that process the framework will
/// check that all children of the [renderBox] have also been disposed.
/// Typically, child [RenderObject]s are disposed by corresponding [Element]s
/// when they are unmounted. However, child render objects that were manually
/// added do not have corresponding [Element]s to manage their lifecycle, and
/// need to be manually disposed here.
///
/// See also:
///
/// * [RenderObjectElement.unmount], which invokes this callback before
/// disposing of its render object.
/// * [RenderObject.dispose], which instructs a render object to release
/// any resources it may be holding.
final VoidCallback? onUnmount;
@override
RenderBox createRenderObject(BuildContext context) => renderBox;
@override
void updateRenderObject(BuildContext context, RenderBox renderObject) {
onBuild?.call();
}
@override
void didUnmountRenderObject(RenderObject renderObject) {
assert(renderObject == renderBox);
onUnmount?.call();
}
}
// EVENT HANDLING
/// A widget that calls callbacks in response to common pointer events.
///
/// It listens to events that can construct gestures, such as when the
/// pointer is pressed, moved, then released or canceled.
///
/// It does not listen to events that are exclusive to mouse, such as when the
/// mouse enters, exits or hovers a region without pressing any buttons. For
/// these events, use [MouseRegion].
///
/// Rather than listening for raw pointer events, consider listening for
/// higher-level gestures using [GestureDetector].
///
/// ## Layout behavior
///
/// _See [BoxConstraints] for an introduction to box layout models._
///
/// If it has a child, this widget defers to the child for sizing behavior. If
/// it does not have a child, it grows to fit the parent instead.
///
/// {@tool dartpad}
/// This example makes a [Container] react to being touched, showing a count of
/// the number of pointer downs and ups.
///
/// ** See code in examples/api/lib/widgets/basic/listener.0.dart **
/// {@end-tool}
class Listener extends SingleChildRenderObjectWidget {
/// Creates a widget that forwards point events to callbacks.
///
/// The [behavior] argument defaults to [HitTestBehavior.deferToChild].
const Listener({
super.key,
this.onPointerDown,
this.onPointerMove,
this.onPointerUp,
this.onPointerHover,
this.onPointerCancel,
this.onPointerPanZoomStart,
this.onPointerPanZoomUpdate,
this.onPointerPanZoomEnd,
this.onPointerSignal,
this.behavior = HitTestBehavior.deferToChild,
super.child,
});
/// Called when a pointer comes into contact with the screen (for touch
/// pointers), or has its button pressed (for mouse pointers) at this widget's
/// location.
final PointerDownEventListener? onPointerDown;
/// Called when a pointer that triggered an [onPointerDown] changes position.
final PointerMoveEventListener? onPointerMove;
/// Called when a pointer that triggered an [onPointerDown] is no longer in
/// contact with the screen.
final PointerUpEventListener? onPointerUp;
/// Called when a pointer that has not triggered an [onPointerDown] changes
/// position.
///
/// This is only fired for pointers which report their location when not down
/// (e.g. mouse pointers, but not most touch pointers).
final PointerHoverEventListener? onPointerHover;
/// Called when the input from a pointer that triggered an [onPointerDown] is
/// no longer directed towards this receiver.
final PointerCancelEventListener? onPointerCancel;
/// Called when a pan/zoom begins such as from a trackpad gesture.
final PointerPanZoomStartEventListener? onPointerPanZoomStart;
/// Called when a pan/zoom is updated.
final PointerPanZoomUpdateEventListener? onPointerPanZoomUpdate;
/// Called when a pan/zoom finishes.
final PointerPanZoomEndEventListener? onPointerPanZoomEnd;
/// Called when a pointer signal occurs over this object.
///
/// See also:
///
/// * [PointerSignalEvent], which goes into more detail on pointer signal
/// events.
final PointerSignalEventListener? onPointerSignal;
/// How to behave during hit testing.
final HitTestBehavior behavior;
@override
RenderPointerListener createRenderObject(BuildContext context) {
return RenderPointerListener(
onPointerDown: onPointerDown,
onPointerMove: onPointerMove,
onPointerUp: onPointerUp,
onPointerHover: onPointerHover,
onPointerCancel: onPointerCancel,
onPointerPanZoomStart: onPointerPanZoomStart,
onPointerPanZoomUpdate: onPointerPanZoomUpdate,
onPointerPanZoomEnd: onPointerPanZoomEnd,
onPointerSignal: onPointerSignal,
behavior: behavior,
);
}
@override
void updateRenderObject(BuildContext context, RenderPointerListener renderObject) {
renderObject
..onPointerDown = onPointerDown
..onPointerMove = onPointerMove
..onPointerUp = onPointerUp
..onPointerHover = onPointerHover
..onPointerCancel = onPointerCancel
..onPointerPanZoomStart = onPointerPanZoomStart
..onPointerPanZoomUpdate = onPointerPanZoomUpdate
..onPointerPanZoomEnd = onPointerPanZoomEnd
..onPointerSignal = onPointerSignal
..behavior = behavior;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
final List<String> listeners = <String>[
if (onPointerDown != null) 'down',
if (onPointerMove != null) 'move',
if (onPointerUp != null) 'up',
if (onPointerHover != null) 'hover',
if (onPointerCancel != null) 'cancel',
if (onPointerPanZoomStart != null) 'panZoomStart',
if (onPointerPanZoomUpdate != null) 'panZoomUpdate',
if (onPointerPanZoomEnd != null) 'panZoomEnd',
if (onPointerSignal != null) 'signal',
];
properties.add(IterableProperty<String>('listeners', listeners, ifEmpty: '<none>'));
properties.add(EnumProperty<HitTestBehavior>('behavior', behavior));
}
}
/// A widget that tracks the movement of mice.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=1oF3pI5umck}
///
/// [MouseRegion] is used
/// when it is needed to compare the list of objects that a mouse pointer is
/// hovering over between this frame and the last frame. This means entering
/// events, exiting events, and mouse cursors.
///
/// To listen to general pointer events, use [Listener], or more preferably,
/// [GestureDetector].
///
/// ## Layout behavior
///
/// _See [BoxConstraints] for an introduction to box layout models._
///
/// If it has a child, this widget defers to the child for sizing behavior. If
/// it does not have a child, it grows to fit the parent instead.
///
/// {@tool dartpad}
/// This example makes a [Container] react to being entered by a mouse
/// pointer, showing a count of the number of entries and exits.
///
/// ** See code in examples/api/lib/widgets/basic/mouse_region.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [Listener], a similar widget that tracks pointer events when the pointer
/// has buttons pressed.
class MouseRegion extends SingleChildRenderObjectWidget {
/// Creates a widget that forwards mouse events to callbacks.
///
/// By default, all callbacks are empty, [cursor] is [MouseCursor.defer], and
/// [opaque] is true.
const MouseRegion({
super.key,
this.onEnter,
this.onExit,
this.onHover,
this.cursor = MouseCursor.defer,
this.opaque = true,
this.hitTestBehavior,
super.child,
});
/// Triggered when a mouse pointer has entered this widget.
///
/// This callback is triggered when the pointer, with or without buttons
/// pressed, has started to be contained by the region of this widget. More
/// specifically, the callback is triggered by the following cases:
///
/// * This widget has appeared under a pointer.
/// * This widget has moved to under a pointer.
/// * A new pointer has been added to somewhere within this widget.
/// * An existing pointer has moved into this widget.
///
/// This callback is not always matched by an [onExit]. If the [MouseRegion]
/// is unmounted while being hovered by a pointer, the [onExit] of the widget
/// callback will never called. For more details, see [onExit].
///
/// {@template flutter.widgets.MouseRegion.onEnter.triggerTime}
/// The time that this callback is triggered is always between frames: either
/// during the post-frame callbacks, or during the callback of a pointer
/// event.
/// {@endtemplate}
///
/// See also:
///
/// * [onExit], which is triggered when a mouse pointer exits the region.
/// * [MouseTrackerAnnotation.onEnter], which is how this callback is
/// internally implemented.
final PointerEnterEventListener? onEnter;
/// Triggered when a pointer moves into a position within this widget without
/// buttons pressed.
///
/// Usually this is only fired for pointers which report their location when
/// not down (e.g. mouse pointers). Certain devices also fire this event on
/// single taps in accessibility mode.
///
/// This callback is not triggered by the movement of the widget.
///
/// The time that this callback is triggered is during the callback of a
/// pointer event, which is always between frames.
///
/// See also:
///
/// * [Listener.onPointerHover], which does the same job. Prefer using
/// [Listener.onPointerHover], since hover events are similar to other regular
/// events.
final PointerHoverEventListener? onHover;
/// Triggered when a mouse pointer has exited this widget when the widget is
/// still mounted.
///
/// This callback is triggered when the pointer, with or without buttons
/// pressed, has stopped being contained by the region of this widget, except
/// when the exit is caused by the disappearance of this widget. More
/// specifically, this callback is triggered by the following cases:
///
/// * A pointer that is hovering this widget has moved away.
/// * A pointer that is hovering this widget has been removed.
/// * This widget, which is being hovered by a pointer, has moved away.
///
/// And is __not__ triggered by the following case:
///
/// * This widget, which is being hovered by a pointer, has disappeared.
///
/// This means that a [MouseRegion.onExit] might not be matched by a
/// [MouseRegion.onEnter].
///
/// This restriction aims to prevent a common misuse: if [State.setState] is
/// called during [MouseRegion.onExit] without checking whether the widget is
/// still mounted, an exception will occur. This is because the callback is
/// triggered during the post-frame phase, at which point the widget has been
/// unmounted. Since [State.setState] is exclusive to widgets, the restriction
/// is specific to [MouseRegion], and does not apply to its lower-level
/// counterparts, [RenderMouseRegion] and [MouseTrackerAnnotation].
///
/// There are a few ways to mitigate this restriction:
///
/// * If the hover state is completely contained within a widget that
/// unconditionally creates this [MouseRegion], then this will not be a
/// concern, since after the [MouseRegion] is unmounted the state is no
/// longer used.
/// * Otherwise, the outer widget very likely has access to the variable that
/// controls whether this [MouseRegion] is present. If so, call [onExit] at
/// the event that turns the condition from true to false.
/// * In cases where the solutions above won't work, you can always
/// override [State.dispose] and call [onExit], or create your own widget
/// using [RenderMouseRegion].
///
/// {@tool dartpad}
/// The following example shows a blue rectangular that turns yellow when
/// hovered. Since the hover state is completely contained within a widget
/// that unconditionally creates the `MouseRegion`, you can ignore the
/// aforementioned restriction.
///
/// ** See code in examples/api/lib/widgets/basic/mouse_region.on_exit.0.dart **
/// {@end-tool}
///
/// {@tool dartpad}
/// The following example shows a widget that hides its content one second
/// after being hovered, and also exposes the enter and exit callbacks.
/// Because the widget conditionally creates the `MouseRegion`, and leaks the
/// hover state, it needs to take the restriction into consideration. In this
/// case, since it has access to the event that triggers the disappearance of
/// the `MouseRegion`, it triggers the exit callback during that event
/// as well.
///
/// ** See code in examples/api/lib/widgets/basic/mouse_region.on_exit.1.dart **
/// {@end-tool}
///
/// {@macro flutter.widgets.MouseRegion.onEnter.triggerTime}
///
/// See also:
///
/// * [onEnter], which is triggered when a mouse pointer enters the region.
/// * [RenderMouseRegion] and [MouseTrackerAnnotation.onExit], which are how
/// this callback is internally implemented, but without the restriction.
final PointerExitEventListener? onExit;
/// The mouse cursor for mouse pointers that are hovering over the region.
///
/// When a mouse enters the region, its cursor will be changed to the [cursor].
/// When the mouse leaves the region, the cursor will be decided by the region
/// found at the new location.
///
/// The [cursor] defaults to [MouseCursor.defer], deferring the choice of
/// cursor to the next region behind it in hit-test order.
final MouseCursor cursor;
/// Whether this widget should prevent other [MouseRegion]s visually behind it
/// from detecting the pointer.
///
/// This changes the list of regions that a pointer hovers, thus affecting how
/// their [onHover], [onEnter], [onExit], and [cursor] behave.
///
/// If [opaque] is true, this widget will absorb the mouse pointer and
/// prevent this widget's siblings (or any other widgets that are not
/// ancestors or descendants of this widget) from detecting the mouse
/// pointer even when the pointer is within their areas.
///
/// If [opaque] is false, this object will not affect how [MouseRegion]s
/// behind it behave, which will detect the mouse pointer as long as the
/// pointer is within their areas.
///
/// This defaults to true.
final bool opaque;
/// How to behave during hit testing.
///
/// This defaults to [HitTestBehavior.opaque] if null.
final HitTestBehavior? hitTestBehavior;
@override
RenderMouseRegion createRenderObject(BuildContext context) {
return RenderMouseRegion(
onEnter: onEnter,
onHover: onHover,
onExit: onExit,
cursor: cursor,
opaque: opaque,
hitTestBehavior: hitTestBehavior,
);
}
@override
void updateRenderObject(BuildContext context, RenderMouseRegion renderObject) {
renderObject
..onEnter = onEnter
..onHover = onHover
..onExit = onExit
..cursor = cursor
..opaque = opaque
..hitTestBehavior = hitTestBehavior;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
final List<String> listeners = <String>[];
if (onEnter != null) {
listeners.add('enter');
}
if (onExit != null) {
listeners.add('exit');
}
if (onHover != null) {
listeners.add('hover');
}
properties.add(IterableProperty<String>('listeners', listeners, ifEmpty: '<none>'));
properties.add(DiagnosticsProperty<MouseCursor>('cursor', cursor, defaultValue: null));
properties.add(DiagnosticsProperty<bool>('opaque', opaque, defaultValue: true));
}
}
/// A widget that creates a separate display list for its child.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=cVAGLDuc2xE}
///
/// This widget creates a separate display list for its child, which
/// can improve performance if the subtree repaints at different times than
/// the surrounding parts of the tree.
///
/// This is useful since [RenderObject.paint] may be triggered even if its
/// associated [Widget] instances did not change or rebuild. A [RenderObject]
/// will repaint whenever any [RenderObject] that shares the same [Layer] is
/// marked as being dirty and needing paint (see [RenderObject.markNeedsPaint]),
/// such as when an ancestor scrolls or when an ancestor or descendant animates.
///
/// Containing [RenderObject.paint] to parts of the render subtree that are
/// actually visually changing using [RepaintBoundary] explicitly or implicitly
/// is therefore critical to minimizing redundant work and improving the app's
/// performance.
///
/// When a [RenderObject] is flagged as needing to paint via
/// [RenderObject.markNeedsPaint], the nearest ancestor [RenderObject] with
/// [RenderObject.isRepaintBoundary], up to possibly the root of the application,
/// is requested to repaint. That nearest ancestor's [RenderObject.paint] method
/// will cause _all_ of its descendant [RenderObject]s to repaint in the same
/// layer.
///
/// [RepaintBoundary] is therefore used, both while propagating the
/// `markNeedsPaint` flag up the render tree and while traversing down the
/// render tree via [PaintingContext.paintChild], to strategically contain
/// repaints to the render subtree that visually changed for performance. This
/// is done because the [RepaintBoundary] widget creates a [RenderObject] that
/// always has a [Layer], decoupling ancestor render objects from the descendant
/// render objects.
///
/// [RepaintBoundary] has the further side-effect of possibly hinting to the
/// engine that it should further optimize animation performance if the render
/// subtree behind the [RepaintBoundary] is sufficiently complex and is static
/// while the surrounding tree changes frequently. In those cases, the engine
/// may choose to pay a one time cost of rasterizing and caching the pixel
/// values of the subtree for faster future GPU re-rendering speed.
///
/// Several framework widgets insert [RepaintBoundary] widgets to mark natural
/// separation points in applications. For instance, contents in Material Design
/// drawers typically don't change while the drawer opens and closes, so
/// repaints are automatically contained to regions inside or outside the drawer
/// when using the [Drawer] widget during transitions.
///
/// See also:
///
/// * [debugRepaintRainbowEnabled], a debugging flag to help visually monitor
/// render tree repaints in a running app.
/// * [debugProfilePaintsEnabled], a debugging flag to show render tree
/// repaints in the observatory's timeline view.
class RepaintBoundary extends SingleChildRenderObjectWidget {
/// Creates a widget that isolates repaints.
const RepaintBoundary({ super.key, super.child });
/// Wraps the given child in a [RepaintBoundary].
///
/// The key for the [RepaintBoundary] is derived either from the child's key
/// (if the child has a non-null key) or from the given `childIndex`.
RepaintBoundary.wrap(Widget child, int childIndex)
: super(key: ValueKey<Object>(child.key ?? childIndex), child: child);
/// Wraps each of the given children in [RepaintBoundary]s.
///
/// The key for each [RepaintBoundary] is derived either from the wrapped
/// child's key (if the wrapped child has a non-null key) or from the wrapped
/// child's index in the list.
static List<RepaintBoundary> wrapAll(List<Widget> widgets) => <RepaintBoundary>[
for (int i = 0; i < widgets.length; ++i) RepaintBoundary.wrap(widgets[i], i),
];
@override
RenderRepaintBoundary createRenderObject(BuildContext context) => RenderRepaintBoundary();
}
/// A widget that is invisible during hit testing.
///
/// When [ignoring] is true, this widget (and its subtree) is invisible
/// to hit testing. It still consumes space during layout and paints its child
/// as usual. It just cannot be the target of located events, because it returns
/// false from [RenderBox.hitTest].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=qV9pqHWxYgI}
///
/// {@tool dartpad}
/// The following sample has an [IgnorePointer] widget wrapping the `Column`
/// which contains a button.
/// When [ignoring] is set to `true` anything inside the `Column` can
/// not be tapped. When [ignoring] is set to `false` anything
/// inside the `Column` can be tapped.
///
/// ** See code in examples/api/lib/widgets/basic/ignore_pointer.0.dart **
/// {@end-tool}
///
/// ## Semantics
///
/// Using this class may also affect how the semantics subtree underneath is
/// collected.
///
/// {@template flutter.widgets.IgnorePointer.semantics}
/// If [ignoring] is true, pointer-related [SemanticsAction]s are removed from
/// the semantics subtree. Otherwise, the subtree remains untouched.
/// {@endtemplate}
///
/// {@template flutter.widgets.IgnorePointer.ignoringSemantics}
/// The usages of [ignoringSemantics] are deprecated and not recommended. This
/// property was introduced to workaround the semantics behavior of the
/// [IgnorePointer] and its friends before v3.8.0-12.0.pre.
///
/// Before that version, entire semantics subtree is dropped if [ignoring] is
/// true. Developers can only use [ignoringSemantics] to preserver the semantics
/// subtrees.
///
/// After that version, with [ignoring] set to true, it only prevents semantics
/// user actions in the semantics subtree but leaves the other
/// [SemanticsProperties] intact. Therefore, the [ignoringSemantics] is no
/// longer needed.
///
/// If [ignoringSemantics] is true, the semantics subtree is dropped. Therefore,
/// the subtree will be invisible to assistive technologies.
///
/// If [ignoringSemantics] is false, the semantics subtree is collected as
/// usual.
/// {@endtemplate}
///
/// See also:
///
/// * [AbsorbPointer], which also prevents its children from receiving pointer
/// events but is itself visible to hit testing.
/// * [SliverIgnorePointer], the sliver version of this widget.
class IgnorePointer extends SingleChildRenderObjectWidget {
/// Creates a widget that is invisible to hit testing.
const IgnorePointer({
super.key,
this.ignoring = true,
@Deprecated(
'Use ExcludeSemantics or create a custom ignore pointer widget instead. '
'This feature was deprecated after v3.8.0-12.0.pre.'
)
this.ignoringSemantics,
super.child,
});
/// Whether this widget is ignored during hit testing.
///
/// Regardless of whether this widget is ignored during hit testing, it will
/// still consume space during layout and be visible during painting.
///
/// {@macro flutter.widgets.IgnorePointer.semantics}
///
/// Defaults to true.
final bool ignoring;
/// Whether the semantics of this widget is ignored when compiling the
/// semantics subtree.
///
/// {@macro flutter.widgets.IgnorePointer.ignoringSemantics}
///
/// See [SemanticsNode] for additional information about the semantics tree.
@Deprecated(
'Use ExcludeSemantics or create a custom ignore pointer widget instead. '
'This feature was deprecated after v3.8.0-12.0.pre.'
)
final bool? ignoringSemantics;
@override
RenderIgnorePointer createRenderObject(BuildContext context) {
return RenderIgnorePointer(
ignoring: ignoring,
ignoringSemantics: ignoringSemantics,
);
}
@override
void updateRenderObject(BuildContext context, RenderIgnorePointer renderObject) {
renderObject
..ignoring = ignoring
..ignoringSemantics = ignoringSemantics;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<bool>('ignoring', ignoring));
properties.add(DiagnosticsProperty<bool>('ignoringSemantics', ignoringSemantics, defaultValue: null));
}
}
/// A widget that absorbs pointers during hit testing.
///
/// When [absorbing] is true, this widget prevents its subtree from receiving
/// pointer events by terminating hit testing at itself. It still consumes space
/// during layout and paints its child as usual. It just prevents its children
/// from being the target of located events, because it returns true from
/// [RenderBox.hitTest].
///
/// When [ignoringSemantics] is true, the subtree will be invisible to
/// the semantics layer (and thus e.g. accessibility tools).
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=65HoWqBboI8}
///
/// {@tool dartpad}
/// The following sample has an [AbsorbPointer] widget wrapping the button on
/// top of the stack, which absorbs pointer events, preventing its child button
/// __and__ the button below it in the stack from receiving the pointer events.
///
/// ** See code in examples/api/lib/widgets/basic/absorb_pointer.0.dart **
/// {@end-tool}
///
/// ## Semantics
///
/// Using this class may also affect how the semantics subtree underneath is
/// collected.
///
/// {@template flutter.widgets.AbsorbPointer.semantics}
/// If [absorbing] is true, pointer-related [SemanticsAction]s are removed from
/// the semantics subtree. Otherwise, the subtree remains untouched.
/// {@endtemplate}
///
/// {@template flutter.widgets.AbsorbPointer.ignoringSemantics}
/// The usages of [ignoringSemantics] are deprecated and not recommended. This
/// property was introduced to workaround the semantics behavior of the
/// [IgnorePointer] and its friends before v3.8.0-12.0.pre.
///
/// Before that version, entire semantics subtree is dropped if [absorbing] is
/// true. Developers can only use [ignoringSemantics] to preserver the semantics
/// subtrees.
///
/// After that version, with [absorbing] set to true, it only prevents semantics
/// user actions in the semantics subtree but leaves the other
/// [SemanticsProperties] intact. Therefore, the [ignoringSemantics] is no
/// longer needed.
///
/// If [ignoringSemantics] is true, the semantics subtree is dropped. Therefore,
/// the subtree will be invisible to assistive technologies.
///
/// If [ignoringSemantics] is false, the semantics subtree is collected as
/// usual.
/// {@endtemplate}
///
/// See also:
///
/// * [IgnorePointer], which also prevents its children from receiving pointer
/// events but is itself invisible to hit testing.
class AbsorbPointer extends SingleChildRenderObjectWidget {
/// Creates a widget that absorbs pointers during hit testing.
const AbsorbPointer({
super.key,
this.absorbing = true,
@Deprecated(
'Use ExcludeSemantics or create a custom absorb pointer widget instead. '
'This feature was deprecated after v3.8.0-12.0.pre.'
)
this.ignoringSemantics,
super.child,
});
/// Whether this widget absorbs pointers during hit testing.
///
/// Regardless of whether this render object absorbs pointers during hit
/// testing, it will still consume space during layout and be visible during
/// painting.
///
/// {@macro flutter.widgets.AbsorbPointer.semantics}
///
/// Defaults to true.
final bool absorbing;
/// Whether the semantics of this render object is ignored when compiling the
/// semantics tree.
///
/// {@macro flutter.widgets.AbsorbPointer.ignoringSemantics}
///
/// See [SemanticsNode] for additional information about the semantics tree.
@Deprecated(
'Use ExcludeSemantics or create a custom absorb pointer widget instead. '
'This feature was deprecated after v3.8.0-12.0.pre.'
)
final bool? ignoringSemantics;
@override
RenderAbsorbPointer createRenderObject(BuildContext context) {
return RenderAbsorbPointer(
absorbing: absorbing,
ignoringSemantics: ignoringSemantics,
);
}
@override
void updateRenderObject(BuildContext context, RenderAbsorbPointer renderObject) {
renderObject
..absorbing = absorbing
..ignoringSemantics = ignoringSemantics;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<bool>('absorbing', absorbing));
properties.add(DiagnosticsProperty<bool>('ignoringSemantics', ignoringSemantics, defaultValue: null));
}
}
/// Holds opaque meta data in the render tree.
///
/// Useful for decorating the render tree with information that will be consumed
/// later. For example, you could store information in the render tree that will
/// be used when the user interacts with the render tree but has no visual
/// impact prior to the interaction.
class MetaData extends SingleChildRenderObjectWidget {
/// Creates a widget that hold opaque meta data.
///
/// The [behavior] argument defaults to [HitTestBehavior.deferToChild].
const MetaData({
super.key,
this.metaData,
this.behavior = HitTestBehavior.deferToChild,
super.child,
});
/// Opaque meta data ignored by the render tree.
final dynamic metaData;
/// How to behave during hit testing.
final HitTestBehavior behavior;
@override
RenderMetaData createRenderObject(BuildContext context) {
return RenderMetaData(
metaData: metaData,
behavior: behavior,
);
}
@override
void updateRenderObject(BuildContext context, RenderMetaData renderObject) {
renderObject
..metaData = metaData
..behavior = behavior;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<HitTestBehavior>('behavior', behavior));
properties.add(DiagnosticsProperty<dynamic>('metaData', metaData));
}
}
// UTILITY NODES
/// A widget that annotates the widget tree with a description of the meaning of
/// the widgets.
///
/// Used by assistive technologies, search engines, and other semantic analysis
/// software to determine the meaning of the application.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=NvtMt_DtFrQ}
///
/// See also:
///
/// * [SemanticsProperties], which contains a complete documentation for each
/// of the constructor parameters that belongs to semantics properties.
/// * [MergeSemantics], which marks a subtree as being a single node for
/// accessibility purposes.
/// * [ExcludeSemantics], which excludes a subtree from the semantics tree
/// (which might be useful if it is, e.g., totally decorative and not
/// important to the user).
/// * [RenderObject.describeSemanticsConfiguration], the rendering library API
/// through which the [Semantics] widget is actually implemented.
/// * [SemanticsNode], the object used by the rendering library to represent
/// semantics in the semantics tree.
/// * [SemanticsDebugger], an overlay to help visualize the semantics tree. Can
/// be enabled using [WidgetsApp.showSemanticsDebugger],
/// [MaterialApp.showSemanticsDebugger], or [CupertinoApp.showSemanticsDebugger].
@immutable
class Semantics extends SingleChildRenderObjectWidget {
/// Creates a semantic annotation.
///
/// To create a `const` instance of [Semantics], use the
/// [Semantics.fromProperties] constructor.
///
/// See also:
///
/// * [SemanticsProperties], which contains a complete documentation for each
/// of the constructor parameters that belongs to semantics properties.
/// * [SemanticsSortKey] for a class that determines accessibility traversal
/// order.
Semantics({
Key? key,
Widget? child,
bool container = false,
bool explicitChildNodes = false,
bool excludeSemantics = false,
bool blockUserActions = false,
bool? enabled,
bool? checked,
bool? mixed,
bool? selected,
bool? toggled,
bool? button,
bool? slider,
bool? keyboardKey,
bool? link,
bool? header,
bool? textField,
bool? readOnly,
bool? focusable,
bool? focused,
bool? inMutuallyExclusiveGroup,
bool? obscured,
bool? multiline,
bool? scopesRoute,
bool? namesRoute,
bool? hidden,
bool? image,
bool? liveRegion,
bool? expanded,
int? maxValueLength,
int? currentValueLength,
String? identifier,
String? label,
AttributedString? attributedLabel,
String? value,
AttributedString? attributedValue,
String? increasedValue,
AttributedString? attributedIncreasedValue,
String? decreasedValue,
AttributedString? attributedDecreasedValue,
String? hint,
AttributedString? attributedHint,
String? tooltip,
String? onTapHint,
String? onLongPressHint,
TextDirection? textDirection,
SemanticsSortKey? sortKey,
SemanticsTag? tagForChildren,
VoidCallback? onTap,
VoidCallback? onLongPress,
VoidCallback? onScrollLeft,
VoidCallback? onScrollRight,
VoidCallback? onScrollUp,
VoidCallback? onScrollDown,
VoidCallback? onIncrease,
VoidCallback? onDecrease,
VoidCallback? onCopy,
VoidCallback? onCut,
VoidCallback? onPaste,
VoidCallback? onDismiss,
MoveCursorHandler? onMoveCursorForwardByCharacter,
MoveCursorHandler? onMoveCursorBackwardByCharacter,
SetSelectionHandler? onSetSelection,
SetTextHandler? onSetText,
VoidCallback? onDidGainAccessibilityFocus,
VoidCallback? onDidLoseAccessibilityFocus,
Map<CustomSemanticsAction, VoidCallback>? customSemanticsActions,
}) : this.fromProperties(
key: key,
child: child,
container: container,
explicitChildNodes: explicitChildNodes,
excludeSemantics: excludeSemantics,
blockUserActions: blockUserActions,
properties: SemanticsProperties(
enabled: enabled,
checked: checked,
mixed: mixed,
expanded: expanded,
toggled: toggled,
selected: selected,
button: button,
slider: slider,
keyboardKey: keyboardKey,
link: link,
header: header,
textField: textField,
readOnly: readOnly,
focusable: focusable,
focused: focused,
inMutuallyExclusiveGroup: inMutuallyExclusiveGroup,
obscured: obscured,
multiline: multiline,
scopesRoute: scopesRoute,
namesRoute: namesRoute,
hidden: hidden,
image: image,
liveRegion: liveRegion,
maxValueLength: maxValueLength,
currentValueLength: currentValueLength,
identifier: identifier,
label: label,
attributedLabel: attributedLabel,
value: value,
attributedValue: attributedValue,
increasedValue: increasedValue,
attributedIncreasedValue: attributedIncreasedValue,
decreasedValue: decreasedValue,
attributedDecreasedValue: attributedDecreasedValue,
hint: hint,
attributedHint: attributedHint,
tooltip: tooltip,
textDirection: textDirection,
sortKey: sortKey,
tagForChildren: tagForChildren,
onTap: onTap,
onLongPress: onLongPress,
onScrollLeft: onScrollLeft,
onScrollRight: onScrollRight,
onScrollUp: onScrollUp,
onScrollDown: onScrollDown,
onIncrease: onIncrease,
onDecrease: onDecrease,
onCopy: onCopy,
onCut: onCut,
onPaste: onPaste,
onMoveCursorForwardByCharacter: onMoveCursorForwardByCharacter,
onMoveCursorBackwardByCharacter: onMoveCursorBackwardByCharacter,
onDidGainAccessibilityFocus: onDidGainAccessibilityFocus,
onDidLoseAccessibilityFocus: onDidLoseAccessibilityFocus,
onDismiss: onDismiss,
onSetSelection: onSetSelection,
onSetText: onSetText,
customSemanticsActions: customSemanticsActions,
hintOverrides: onTapHint != null || onLongPressHint != null ?
SemanticsHintOverrides(
onTapHint: onTapHint,
onLongPressHint: onLongPressHint,
) : null,
),
);
/// Creates a semantic annotation using [SemanticsProperties].
const Semantics.fromProperties({
super.key,
super.child,
this.container = false,
this.explicitChildNodes = false,
this.excludeSemantics = false,
this.blockUserActions = false,
required this.properties,
});
/// Contains properties used by assistive technologies to make the application
/// more accessible.
final SemanticsProperties properties;
/// If [container] is true, this widget will introduce a new
/// node in the semantics tree. Otherwise, the semantics will be
/// merged with the semantics of any ancestors (if the ancestor allows that).
///
/// Whether descendants of this widget can add their semantic information to the
/// [SemanticsNode] introduced by this configuration is controlled by
/// [explicitChildNodes].
final bool container;
/// Whether descendants of this widget are allowed to add semantic information
/// to the [SemanticsNode] annotated by this widget.
///
/// When set to false descendants are allowed to annotate [SemanticsNode]s of
/// their parent with the semantic information they want to contribute to the
/// semantic tree.
/// When set to true the only way for descendants to contribute semantic
/// information to the semantic tree is to introduce new explicit
/// [SemanticsNode]s to the tree.
///
/// If the semantics properties of this node include
/// [SemanticsProperties.scopesRoute] set to true, then [explicitChildNodes]
/// must be true also.
///
/// This setting is often used in combination with [SemanticsConfiguration.isSemanticBoundary]
/// to create semantic boundaries that are either writable or not for children.
final bool explicitChildNodes;
/// Whether to replace all child semantics with this node.
///
/// Defaults to false.
///
/// When this flag is set to true, all child semantics nodes are ignored.
/// This can be used as a convenience for cases where a child is wrapped in
/// an [ExcludeSemantics] widget and then another [Semantics] widget.
final bool excludeSemantics;
/// Whether to block user interactions for the rendering subtree.
///
/// Setting this to true will prevent users from interacting with The
/// rendering object configured by this widget and its subtree through
/// pointer-related [SemanticsAction]s in assistive technologies.
///
/// The [SemanticsNode] created from this widget is still focusable by
/// assistive technologies. Only pointer-related [SemanticsAction]s, such as
/// [SemanticsAction.tap] or its friends, are blocked.
///
/// If this widget is merged into a parent semantics node, only the
/// [SemanticsAction]s of this widget and the widgets in the subtree are
/// blocked.
///
/// For example:
/// ```dart
/// void _myTap() { }
/// void _myLongPress() { }
///
/// Widget build(BuildContext context) {
/// return Semantics(
/// onTap: _myTap,
/// child: Semantics(
/// blockUserActions: true,
/// onLongPress: _myLongPress,
/// child: const Text('label'),
/// ),
/// );
/// }
/// ```
///
/// The result semantics node will still have `_myTap`, but the `_myLongPress`
/// will be blocked.
final bool blockUserActions;
@override
RenderSemanticsAnnotations createRenderObject(BuildContext context) {
return RenderSemanticsAnnotations(
container: container,
explicitChildNodes: explicitChildNodes,
excludeSemantics: excludeSemantics,
blockUserActions: blockUserActions,
properties: properties,
textDirection: _getTextDirection(context),
);
}
TextDirection? _getTextDirection(BuildContext context) {
if (properties.textDirection != null) {
return properties.textDirection;
}
final bool containsText = properties.attributedLabel != null ||
properties.label != null ||
properties.value != null ||
properties.hint != null ||
properties.tooltip != null;
if (!containsText) {
return null;
}
return Directionality.maybeOf(context);
}
@override
void updateRenderObject(BuildContext context, RenderSemanticsAnnotations renderObject) {
renderObject
..container = container
..explicitChildNodes = explicitChildNodes
..excludeSemantics = excludeSemantics
..blockUserActions = blockUserActions
..properties = properties
..textDirection = _getTextDirection(context);
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<bool>('container', container));
properties.add(DiagnosticsProperty<SemanticsProperties>('properties', this.properties));
this.properties.debugFillProperties(properties);
}
}
/// A widget that merges the semantics of its descendants.
///
/// Causes all the semantics of the subtree rooted at this node to be
/// merged into one node in the semantics tree. For example, if you
/// have a widget with a Text node next to a checkbox widget, this
/// could be used to merge the label from the Text node with the
/// "checked" semantic state of the checkbox into a single node that
/// had both the label and the checked state. Otherwise, the label
/// would be presented as a separate feature than the checkbox, and
/// the user would not be able to be sure that they were related.
///
/// {@tool snippet}
/// This snippet shows how to use [MergeSemantics] to merge the semantics of
/// a [Checkbox] and [Text] widget.
///
/// ```dart
/// MergeSemantics(
/// child: Row(
/// children: <Widget>[
/// Checkbox(
/// value: true,
/// onChanged: (bool? value) {},
/// ),
/// const Text('Settings'),
/// ],
/// ),
/// )
/// ```
/// {@end-tool}
///
/// Be aware that if two nodes in the subtree have conflicting
/// semantics, the result may be nonsensical. For example, a subtree
/// with a checked checkbox and an unchecked checkbox will be
/// presented as checked. All the labels will be merged into a single
/// string (with newlines separating each label from the other). If
/// multiple nodes in the merged subtree can handle semantic gestures,
/// the first one in tree order will be the one to receive the
/// callbacks.
class MergeSemantics extends SingleChildRenderObjectWidget {
/// Creates a widget that merges the semantics of its descendants.
const MergeSemantics({ super.key, super.child });
@override
RenderMergeSemantics createRenderObject(BuildContext context) => RenderMergeSemantics();
}
/// A widget that drops the semantics of all widget that were painted before it
/// in the same semantic container.
///
/// This is useful to hide widgets from accessibility tools that are painted
/// behind a certain widget, e.g. an alert should usually disallow interaction
/// with any widget located "behind" the alert (even when they are still
/// partially visible). Similarly, an open [Drawer] blocks interactions with
/// any widget outside the drawer.
///
/// See also:
///
/// * [ExcludeSemantics] which drops all semantics of its descendants.
class BlockSemantics extends SingleChildRenderObjectWidget {
/// Creates a widget that excludes the semantics of all widgets painted before
/// it in the same semantic container.
const BlockSemantics({ super.key, this.blocking = true, super.child });
/// Whether this widget is blocking semantics of all widget that were painted
/// before it in the same semantic container.
final bool blocking;
@override
RenderBlockSemantics createRenderObject(BuildContext context) => RenderBlockSemantics(blocking: blocking);
@override
void updateRenderObject(BuildContext context, RenderBlockSemantics renderObject) {
renderObject.blocking = blocking;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<bool>('blocking', blocking));
}
}
/// A widget that drops all the semantics of its descendants.
///
/// When [excluding] is true, this widget (and its subtree) is excluded from
/// the semantics tree.
///
/// This can be used to hide descendant widgets that would otherwise be
/// reported but that would only be confusing. For example, the
/// material library's [Chip] widget hides the avatar since it is
/// redundant with the chip label.
///
/// See also:
///
/// * [BlockSemantics] which drops semantics of widgets earlier in the tree.
class ExcludeSemantics extends SingleChildRenderObjectWidget {
/// Creates a widget that drops all the semantics of its descendants.
const ExcludeSemantics({
super.key,
this.excluding = true,
super.child,
});
/// Whether this widget is excluded in the semantics tree.
final bool excluding;
@override
RenderExcludeSemantics createRenderObject(BuildContext context) => RenderExcludeSemantics(excluding: excluding);
@override
void updateRenderObject(BuildContext context, RenderExcludeSemantics renderObject) {
renderObject.excluding = excluding;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<bool>('excluding', excluding));
}
}
/// A widget that annotates the child semantics with an index.
///
/// Semantic indexes are used by TalkBack/Voiceover to make announcements about
/// the current scroll state. Certain widgets like the [ListView] will
/// automatically provide a child index for building semantics. A user may wish
/// to manually provide semantic indexes if not all child of the scrollable
/// contribute semantics.
///
/// {@tool snippet}
///
/// The example below handles spacers in a scrollable that don't contribute
/// semantics. The automatic indexes would give the spaces a semantic index,
/// causing scroll announcements to erroneously state that there are four items
/// visible.
///
/// ```dart
/// ListView(
/// addSemanticIndexes: false,
/// semanticChildCount: 2,
/// children: const <Widget>[
/// IndexedSemantics(index: 0, child: Text('First')),
/// Spacer(),
/// IndexedSemantics(index: 1, child: Text('Second')),
/// Spacer(),
/// ],
/// )
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [CustomScrollView], for an explanation of index semantics.
class IndexedSemantics extends SingleChildRenderObjectWidget {
/// Creates a widget that annotated the first child semantics node with an index.
const IndexedSemantics({
super.key,
required this.index,
super.child,
});
/// The index used to annotate the first child semantics node.
final int index;
@override
RenderIndexedSemantics createRenderObject(BuildContext context) => RenderIndexedSemantics(index: index);
@override
void updateRenderObject(BuildContext context, RenderIndexedSemantics renderObject) {
renderObject.index = index;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<int>('index', index));
}
}
/// A widget that builds its child.
///
/// Useful for attaching a key to an existing widget.
class KeyedSubtree extends StatelessWidget {
/// Creates a widget that builds its child.
const KeyedSubtree({super.key, required this.child});
/// Creates a KeyedSubtree for child with a key that's based on the child's existing key or childIndex.
KeyedSubtree.wrap(this.child, int childIndex)
: super(key: ValueKey<Object>(child.key ?? childIndex));
/// The widget below this widget in the tree.
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// Wrap each item in a KeyedSubtree whose key is based on the item's existing key or
/// the sum of its list index and `baseIndex`.
static List<Widget> ensureUniqueKeysForList(List<Widget> items, { int baseIndex = 0 }) {
if (items.isEmpty) {
return items;
}
final List<Widget> itemsWithUniqueKeys = <Widget>[];
int itemIndex = baseIndex;
for (final Widget item in items) {
itemsWithUniqueKeys.add(KeyedSubtree.wrap(item, itemIndex));
itemIndex += 1;
}
assert(!debugItemsHaveDuplicateKeys(itemsWithUniqueKeys));
return itemsWithUniqueKeys;
}
@override
Widget build(BuildContext context) => child;
}
/// A stateless utility widget whose [build] method uses its
/// [builder] callback to create the widget's child.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=xXNOkIuSYuA}
///
/// This widget is an inline alternative to defining a [StatelessWidget]
/// subclass. For example, instead of defining a widget as follows:
///
/// ```dart
/// class Foo extends StatelessWidget {
/// const Foo({super.key});
/// @override
/// Widget build(BuildContext context) => const Text('foo');
/// }
/// ```
///
/// ...and using it in the usual way:
///
/// ```dart
/// // continuing from previous example...
/// const Center(child: Foo())
/// ```
///
/// ...one could instead define and use it in a single step, without
/// defining a new widget class:
///
/// ```dart
/// Center(
/// child: Builder(
/// builder: (BuildContext context) => const Text('foo'),
/// ),
/// )
/// ```
///
/// The difference between either of the previous examples and
/// creating a child directly without an intervening widget, is the
/// extra [BuildContext] element that the additional widget adds. This
/// is particularly noticeable when the tree contains an inherited
/// widget that is referred to by a method like [Scaffold.of],
/// which visits the child widget's BuildContext ancestors.
///
/// In the following example the button's `onPressed` callback is unable
/// to find the enclosing [ScaffoldState] with [Scaffold.of]:
///
/// ```dart
/// Widget build(BuildContext context) {
/// return Scaffold(
/// body: Center(
/// child: TextButton(
/// onPressed: () {
/// // Fails because Scaffold.of() doesn't find anything
/// // above this widget's context.
/// print(Scaffold.of(context).hasAppBar);
/// },
/// child: const Text('hasAppBar'),
/// )
/// ),
/// );
/// }
/// ```
///
/// A [Builder] widget introduces an additional [BuildContext] element
/// and so the [Scaffold.of] method succeeds.
///
/// ```dart
/// Widget build(BuildContext context) {
/// return Scaffold(
/// body: Builder(
/// builder: (BuildContext context) {
/// return Center(
/// child: TextButton(
/// onPressed: () {
/// print(Scaffold.of(context).hasAppBar);
/// },
/// child: const Text('hasAppBar'),
/// ),
/// );
/// },
/// ),
/// );
/// }
/// ```
///
/// See also:
///
/// * [StatefulBuilder], A stateful utility widget whose [build] method uses its
/// [builder] callback to create the widget's child.
class Builder extends StatelessWidget {
/// Creates a widget that delegates its build to a callback.
const Builder({
super.key,
required this.builder,
});
/// Called to obtain the child widget.
///
/// This function is called whenever this widget is included in its parent's
/// build and the old widget (if any) that it synchronizes with has a distinct
/// object identity. Typically the parent's build method will construct
/// a new tree of widgets and so a new Builder child will not be [identical]
/// to the corresponding old one.
final WidgetBuilder builder;
@override
Widget build(BuildContext context) => builder(context);
}
/// Signature for the builder callback used by [StatefulBuilder].
///
/// Call `setState` to schedule the [StatefulBuilder] to rebuild.
typedef StatefulWidgetBuilder = Widget Function(BuildContext context, StateSetter setState);
/// A platonic widget that both has state and calls a closure to obtain its child widget.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=syvT63CosNE}
///
/// The [StateSetter] function passed to the [builder] is used to invoke a
/// rebuild instead of a typical [State]'s [State.setState].
///
/// Since the [builder] is re-invoked when the [StateSetter] is called, any
/// variables that represents state should be kept outside the [builder] function.
///
/// {@tool snippet}
///
/// This example shows using an inline StatefulBuilder that rebuilds and that
/// also has state.
///
/// ```dart
/// await showDialog<void>(
/// context: context,
/// builder: (BuildContext context) {
/// int? selectedRadio = 0;
/// return AlertDialog(
/// content: StatefulBuilder(
/// builder: (BuildContext context, StateSetter setState) {
/// return Column(
/// mainAxisSize: MainAxisSize.min,
/// children: List<Widget>.generate(4, (int index) {
/// return Radio<int>(
/// value: index,
/// groupValue: selectedRadio,
/// onChanged: (int? value) {
/// setState(() => selectedRadio = value);
/// },
/// );
/// }),
/// );
/// },
/// ),
/// );
/// },
/// );
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [Builder], the platonic stateless widget.
class StatefulBuilder extends StatefulWidget {
/// Creates a widget that both has state and delegates its build to a callback.
const StatefulBuilder({
super.key,
required this.builder,
});
/// Called to obtain the child widget.
///
/// This function is called whenever this widget is included in its parent's
/// build and the old widget (if any) that it synchronizes with has a distinct
/// object identity. Typically the parent's build method will construct
/// a new tree of widgets and so a new Builder child will not be [identical]
/// to the corresponding old one.
final StatefulWidgetBuilder builder;
@override
State<StatefulBuilder> createState() => _StatefulBuilderState();
}
class _StatefulBuilderState extends State<StatefulBuilder> {
@override
Widget build(BuildContext context) => widget.builder(context, setState);
}
/// A widget that paints its area with a specified [Color] and then draws its
/// child on top of that color.
class ColoredBox extends SingleChildRenderObjectWidget {
/// Creates a widget that paints its area with the specified [Color].
const ColoredBox({ required this.color, super.child, super.key });
/// The color to paint the background area with.
final Color color;
@override
RenderObject createRenderObject(BuildContext context) {
return _RenderColoredBox(color: color);
}
@override
void updateRenderObject(BuildContext context, RenderObject renderObject) {
(renderObject as _RenderColoredBox).color = color;
}
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DiagnosticsProperty<Color>('color', color));
}
}
class _RenderColoredBox extends RenderProxyBoxWithHitTestBehavior {
_RenderColoredBox({ required Color color })
: _color = color,
super(behavior: HitTestBehavior.opaque);
/// The fill color for this render object.
Color get color => _color;
Color _color;
set color(Color value) {
if (value == _color) {
return;
}
_color = value;
markNeedsPaint();
}
@override
void paint(PaintingContext context, Offset offset) {
// It's tempting to want to optimize out this `drawRect()` call if the
// color is transparent (alpha==0), but doing so would be incorrect. See
// https://github.com/flutter/flutter/pull/72526#issuecomment-749185938 for
// a good description of why.
if (size > Size.zero) {
context.canvas.drawRect(offset & size, Paint()..color = color);
}
if (child != null) {
context.paintChild(child!, offset);
}
}
}
| flutter/packages/flutter/lib/src/widgets/basic.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/basic.dart",
"repo_id": "flutter",
"token_count": 88727
} | 670 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart' show kIsWeb;
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'basic.dart';
import 'binding.dart';
import 'debug.dart';
import 'framework.dart';
import 'media_query.dart';
import 'overlay.dart';
import 'view.dart';
/// Signature for determining whether the given data will be accepted by a [DragTarget].
///
/// Used by [DragTarget.onWillAccept].
typedef DragTargetWillAccept<T> = bool Function(T? data);
/// Signature for determining whether the given data will be accepted by a [DragTarget],
/// based on provided information.
///
/// Used by [DragTarget.onWillAcceptWithDetails].
typedef DragTargetWillAcceptWithDetails<T> = bool Function(DragTargetDetails<T> details);
/// Signature for causing a [DragTarget] to accept the given data.
///
/// Used by [DragTarget.onAccept].
typedef DragTargetAccept<T> = void Function(T data);
/// Signature for determining information about the acceptance by a [DragTarget].
///
/// Used by [DragTarget.onAcceptWithDetails].
typedef DragTargetAcceptWithDetails<T> = void Function(DragTargetDetails<T> details);
/// Signature for building children of a [DragTarget].
///
/// The `candidateData` argument contains the list of drag data that is hovering
/// over this [DragTarget] and that has passed [DragTarget.onWillAccept]. The
/// `rejectedData` argument contains the list of drag data that is hovering over
/// this [DragTarget] and that will not be accepted by the [DragTarget].
///
/// Used by [DragTarget.builder].
typedef DragTargetBuilder<T> = Widget Function(BuildContext context, List<T?> candidateData, List<dynamic> rejectedData);
/// Signature for when a [Draggable] is dragged across the screen.
///
/// Used by [Draggable.onDragUpdate].
typedef DragUpdateCallback = void Function(DragUpdateDetails details);
/// Signature for when a [Draggable] is dropped without being accepted by a [DragTarget].
///
/// Used by [Draggable.onDraggableCanceled].
typedef DraggableCanceledCallback = void Function(Velocity velocity, Offset offset);
/// Signature for when the draggable is dropped.
///
/// The velocity and offset at which the pointer was moving when the draggable
/// was dropped is available in the [DraggableDetails]. Also included in the
/// `details` is whether the draggable's [DragTarget] accepted it.
///
/// Used by [Draggable.onDragEnd].
typedef DragEndCallback = void Function(DraggableDetails details);
/// Signature for when a [Draggable] leaves a [DragTarget].
///
/// Used by [DragTarget.onLeave].
typedef DragTargetLeave<T> = void Function(T? data);
/// Signature for when a [Draggable] moves within a [DragTarget].
///
/// Used by [DragTarget.onMove].
typedef DragTargetMove<T> = void Function(DragTargetDetails<T> details);
/// Signature for the strategy that determines the drag start point of a [Draggable].
///
/// Used by [Draggable.dragAnchorStrategy].
///
/// There are two built-in strategies:
///
/// * [childDragAnchorStrategy], which displays the feedback anchored at the
/// position of the original child.
///
/// * [pointerDragAnchorStrategy], which displays the feedback anchored at the
/// position of the touch that started the drag.
typedef DragAnchorStrategy = Offset Function(Draggable<Object> draggable, BuildContext context, Offset position);
/// Display the feedback anchored at the position of the original child.
///
/// If feedback is identical to the child, then this means the feedback will
/// exactly overlap the original child when the drag starts.
///
/// This is the default [DragAnchorStrategy].
///
/// See also:
///
/// * [DragAnchorStrategy], the typedef that this function implements.
/// * [Draggable.dragAnchorStrategy], for which this is a built-in value.
Offset childDragAnchorStrategy(Draggable<Object> draggable, BuildContext context, Offset position) {
final RenderBox renderObject = context.findRenderObject()! as RenderBox;
return renderObject.globalToLocal(position);
}
/// Display the feedback anchored at the position of the touch that started
/// the drag.
///
/// If feedback is identical to the child, then this means the top left of the
/// feedback will be under the finger when the drag starts. This will likely not
/// exactly overlap the original child, e.g. if the child is big and the touch
/// was not centered. This mode is useful when the feedback is transformed so as
/// to move the feedback to the left by half its width, and up by half its width
/// plus the height of the finger, since then it appears as if putting the
/// finger down makes the touch feedback appear above the finger. (It feels
/// weird for it to appear offset from the original child if it's anchored to
/// the child and not the finger.)
///
/// See also:
///
/// * [DragAnchorStrategy], the typedef that this function implements.
/// * [Draggable.dragAnchorStrategy], for which this is a built-in value.
Offset pointerDragAnchorStrategy(Draggable<Object> draggable, BuildContext context, Offset position) {
return Offset.zero;
}
/// A widget that can be dragged from to a [DragTarget].
///
/// When a draggable widget recognizes the start of a drag gesture, it displays
/// a [feedback] widget that tracks the user's finger across the screen. If the
/// user lifts their finger while on top of a [DragTarget], that target is given
/// the opportunity to accept the [data] carried by the draggable.
///
/// The [ignoringFeedbackPointer] defaults to true, which means that
/// the [feedback] widget ignores the pointer during hit testing. Similarly,
/// [ignoringFeedbackSemantics] defaults to true, and the [feedback] also ignores
/// semantics when building the semantics tree.
///
/// On multitouch devices, multiple drags can occur simultaneously because there
/// can be multiple pointers in contact with the device at once. To limit the
/// number of simultaneous drags, use the [maxSimultaneousDrags] property. The
/// default is to allow an unlimited number of simultaneous drags.
///
/// This widget displays [child] when zero drags are under way. If
/// [childWhenDragging] is non-null, this widget instead displays
/// [childWhenDragging] when one or more drags are underway. Otherwise, this
/// widget always displays [child].
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=q4x2G_9-Mu0}
///
/// {@tool dartpad}
/// The following example has a [Draggable] widget along with a [DragTarget]
/// in a row demonstrating an incremented `acceptedData` integer value when
/// you drag the element to the target.
///
/// ** See code in examples/api/lib/widgets/drag_target/draggable.0.dart **
/// {@end-tool}
///
/// See also:
///
/// * [DragTarget]
/// * [LongPressDraggable]
class Draggable<T extends Object> extends StatefulWidget {
/// Creates a widget that can be dragged to a [DragTarget].
///
/// If [maxSimultaneousDrags] is non-null, it must be non-negative.
const Draggable({
super.key,
required this.child,
required this.feedback,
this.data,
this.axis,
this.childWhenDragging,
this.feedbackOffset = Offset.zero,
this.dragAnchorStrategy = childDragAnchorStrategy,
this.affinity,
this.maxSimultaneousDrags,
this.onDragStarted,
this.onDragUpdate,
this.onDraggableCanceled,
this.onDragEnd,
this.onDragCompleted,
this.ignoringFeedbackSemantics = true,
this.ignoringFeedbackPointer = true,
this.rootOverlay = false,
this.hitTestBehavior = HitTestBehavior.deferToChild,
this.allowedButtonsFilter,
}) : assert(maxSimultaneousDrags == null || maxSimultaneousDrags >= 0);
/// The data that will be dropped by this draggable.
final T? data;
/// The [Axis] to restrict this draggable's movement, if specified.
///
/// When axis is set to [Axis.horizontal], this widget can only be dragged
/// horizontally. Behavior is similar for [Axis.vertical].
///
/// Defaults to allowing drag on both [Axis.horizontal] and [Axis.vertical].
///
/// When null, allows drag on both [Axis.horizontal] and [Axis.vertical].
///
/// For the direction of gestures this widget competes with to start a drag
/// event, see [Draggable.affinity].
final Axis? axis;
/// The widget below this widget in the tree.
///
/// This widget displays [child] when zero drags are under way. If
/// [childWhenDragging] is non-null, this widget instead displays
/// [childWhenDragging] when one or more drags are underway. Otherwise, this
/// widget always displays [child].
///
/// The [feedback] widget is shown under the pointer when a drag is under way.
///
/// To limit the number of simultaneous drags on multitouch devices, see
/// [maxSimultaneousDrags].
///
/// {@macro flutter.widgets.ProxyWidget.child}
final Widget child;
/// The widget to display instead of [child] when one or more drags are under way.
///
/// If this is null, then this widget will always display [child] (and so the
/// drag source representation will not change while a drag is under
/// way).
///
/// The [feedback] widget is shown under the pointer when a drag is under way.
///
/// To limit the number of simultaneous drags on multitouch devices, see
/// [maxSimultaneousDrags].
final Widget? childWhenDragging;
/// The widget to show under the pointer when a drag is under way.
///
/// See [child] and [childWhenDragging] for information about what is shown
/// at the location of the [Draggable] itself when a drag is under way.
final Widget feedback;
/// The feedbackOffset can be used to set the hit test target point for the
/// purposes of finding a drag target. It is especially useful if the feedback
/// is transformed compared to the child.
final Offset feedbackOffset;
/// A strategy that is used by this draggable to get the anchor offset when it
/// is dragged.
///
/// The anchor offset refers to the distance between the users' fingers and
/// the [feedback] widget when this draggable is dragged.
///
/// This property's value is a function that implements [DragAnchorStrategy].
/// There are two built-in functions that can be used:
///
/// * [childDragAnchorStrategy], which displays the feedback anchored at the
/// position of the original child.
///
/// * [pointerDragAnchorStrategy], which displays the feedback anchored at the
/// position of the touch that started the drag.
///
/// Defaults to [childDragAnchorStrategy].
final DragAnchorStrategy dragAnchorStrategy;
/// Whether the semantics of the [feedback] widget is ignored when building
/// the semantics tree.
///
/// This value should be set to false when the [feedback] widget is intended
/// to be the same object as the [child]. Placing a [GlobalKey] on this
/// widget will ensure semantic focus is kept on the element as it moves in
/// and out of the feedback position.
///
/// Defaults to true.
final bool ignoringFeedbackSemantics;
/// Whether the [feedback] widget is ignored during hit testing.
///
/// Regardless of whether this widget is ignored during hit testing, it will
/// still consume space during layout and be visible during painting.
///
/// Defaults to true.
final bool ignoringFeedbackPointer;
/// Controls how this widget competes with other gestures to initiate a drag.
///
/// If affinity is null, this widget initiates a drag as soon as it recognizes
/// a tap down gesture, regardless of any directionality. If affinity is
/// horizontal (or vertical), then this widget will compete with other
/// horizontal (or vertical, respectively) gestures.
///
/// For example, if this widget is placed in a vertically scrolling region and
/// has horizontal affinity, pointer motion in the vertical direction will
/// result in a scroll and pointer motion in the horizontal direction will
/// result in a drag. Conversely, if the widget has a null or vertical
/// affinity, pointer motion in any direction will result in a drag rather
/// than in a scroll because the draggable widget, being the more specific
/// widget, will out-compete the [Scrollable] for vertical gestures.
///
/// For the directions this widget can be dragged in after the drag event
/// starts, see [Draggable.axis].
final Axis? affinity;
/// How many simultaneous drags to support.
///
/// When null, no limit is applied. Set this to 1 if you want to only allow
/// the drag source to have one item dragged at a time. Set this to 0 if you
/// want to prevent the draggable from actually being dragged.
///
/// If you set this property to 1, consider supplying an "empty" widget for
/// [childWhenDragging] to create the illusion of actually moving [child].
final int? maxSimultaneousDrags;
/// Called when the draggable starts being dragged.
final VoidCallback? onDragStarted;
/// Called when the draggable is dragged.
///
/// This function will only be called while this widget is still mounted to
/// the tree (i.e. [State.mounted] is true), and if this widget has actually moved.
final DragUpdateCallback? onDragUpdate;
/// Called when the draggable is dropped without being accepted by a [DragTarget].
///
/// This function might be called after this widget has been removed from the
/// tree. For example, if a drag was in progress when this widget was removed
/// from the tree and the drag ended up being canceled, this callback will
/// still be called. For this reason, implementations of this callback might
/// need to check [State.mounted] to check whether the state receiving the
/// callback is still in the tree.
final DraggableCanceledCallback? onDraggableCanceled;
/// Called when the draggable is dropped and accepted by a [DragTarget].
///
/// This function might be called after this widget has been removed from the
/// tree. For example, if a drag was in progress when this widget was removed
/// from the tree and the drag ended up completing, this callback will
/// still be called. For this reason, implementations of this callback might
/// need to check [State.mounted] to check whether the state receiving the
/// callback is still in the tree.
final VoidCallback? onDragCompleted;
/// Called when the draggable is dropped.
///
/// The velocity and offset at which the pointer was moving when it was
/// dropped is available in the [DraggableDetails]. Also included in the
/// `details` is whether the draggable's [DragTarget] accepted it.
///
/// This function will only be called while this widget is still mounted to
/// the tree (i.e. [State.mounted] is true).
final DragEndCallback? onDragEnd;
/// Whether the feedback widget will be put on the root [Overlay].
///
/// When false, the feedback widget will be put on the closest [Overlay]. When
/// true, the [feedback] widget will be put on the farthest (aka root)
/// [Overlay].
///
/// Defaults to false.
final bool rootOverlay;
/// How to behave during hit test.
///
/// Defaults to [HitTestBehavior.deferToChild].
final HitTestBehavior hitTestBehavior;
/// {@macro flutter.gestures.multidrag._allowedButtonsFilter}
final AllowedButtonsFilter? allowedButtonsFilter;
/// Creates a gesture recognizer that recognizes the start of the drag.
///
/// Subclasses can override this function to customize when they start
/// recognizing a drag.
@protected
MultiDragGestureRecognizer createRecognizer(GestureMultiDragStartCallback onStart) {
switch (affinity) {
case Axis.horizontal:
return HorizontalMultiDragGestureRecognizer(allowedButtonsFilter: allowedButtonsFilter)..onStart = onStart;
case Axis.vertical:
return VerticalMultiDragGestureRecognizer(allowedButtonsFilter: allowedButtonsFilter)..onStart = onStart;
case null:
return ImmediateMultiDragGestureRecognizer(allowedButtonsFilter: allowedButtonsFilter)..onStart = onStart;
}
}
@override
State<Draggable<T>> createState() => _DraggableState<T>();
}
/// Makes its child draggable starting from long press.
///
/// See also:
///
/// * [Draggable], similar to the [LongPressDraggable] widget but happens immediately.
/// * [DragTarget], a widget that receives data when a [Draggable] widget is dropped.
class LongPressDraggable<T extends Object> extends Draggable<T> {
/// Creates a widget that can be dragged starting from long press.
///
/// If [maxSimultaneousDrags] is non-null, it must be non-negative.
const LongPressDraggable({
super.key,
required super.child,
required super.feedback,
super.data,
super.axis,
super.childWhenDragging,
super.feedbackOffset,
super.dragAnchorStrategy,
super.maxSimultaneousDrags,
super.onDragStarted,
super.onDragUpdate,
super.onDraggableCanceled,
super.onDragEnd,
super.onDragCompleted,
this.hapticFeedbackOnStart = true,
super.ignoringFeedbackSemantics,
super.ignoringFeedbackPointer,
this.delay = kLongPressTimeout,
super.allowedButtonsFilter,
});
/// Whether haptic feedback should be triggered on drag start.
final bool hapticFeedbackOnStart;
/// The duration that a user has to press down before a long press is registered.
///
/// Defaults to [kLongPressTimeout].
final Duration delay;
@override
DelayedMultiDragGestureRecognizer createRecognizer(GestureMultiDragStartCallback onStart) {
return DelayedMultiDragGestureRecognizer(delay: delay, allowedButtonsFilter: allowedButtonsFilter)
..onStart = (Offset position) {
final Drag? result = onStart(position);
if (result != null && hapticFeedbackOnStart) {
HapticFeedback.selectionClick();
}
return result;
};
}
}
class _DraggableState<T extends Object> extends State<Draggable<T>> {
@override
void initState() {
super.initState();
_recognizer = widget.createRecognizer(_startDrag);
}
@override
void dispose() {
_disposeRecognizerIfInactive();
super.dispose();
}
@override
void didChangeDependencies() {
_recognizer!.gestureSettings = MediaQuery.maybeGestureSettingsOf(context);
super.didChangeDependencies();
}
// This gesture recognizer has an unusual lifetime. We want to support the use
// case of removing the Draggable from the tree in the middle of a drag. That
// means we need to keep this recognizer alive after this state object has
// been disposed because it's the one listening to the pointer events that are
// driving the drag.
//
// We achieve that by keeping count of the number of active drags and only
// disposing the gesture recognizer after (a) this state object has been
// disposed and (b) there are no more active drags.
GestureRecognizer? _recognizer;
int _activeCount = 0;
void _disposeRecognizerIfInactive() {
if (_activeCount > 0) {
return;
}
_recognizer!.dispose();
_recognizer = null;
}
void _routePointer(PointerDownEvent event) {
if (widget.maxSimultaneousDrags != null && _activeCount >= widget.maxSimultaneousDrags!) {
return;
}
_recognizer!.addPointer(event);
}
_DragAvatar<T>? _startDrag(Offset position) {
if (widget.maxSimultaneousDrags != null && _activeCount >= widget.maxSimultaneousDrags!) {
return null;
}
final Offset dragStartPoint;
dragStartPoint = widget.dragAnchorStrategy(widget, context, position);
setState(() {
_activeCount += 1;
});
final _DragAvatar<T> avatar = _DragAvatar<T>(
overlayState: Overlay.of(context, debugRequiredFor: widget, rootOverlay: widget.rootOverlay),
data: widget.data,
axis: widget.axis,
initialPosition: position,
dragStartPoint: dragStartPoint,
feedback: widget.feedback,
feedbackOffset: widget.feedbackOffset,
ignoringFeedbackSemantics: widget.ignoringFeedbackSemantics,
ignoringFeedbackPointer: widget.ignoringFeedbackPointer,
viewId: View.of(context).viewId,
onDragUpdate: (DragUpdateDetails details) {
if (mounted && widget.onDragUpdate != null) {
widget.onDragUpdate!(details);
}
},
onDragEnd: (Velocity velocity, Offset offset, bool wasAccepted) {
if (mounted) {
setState(() {
_activeCount -= 1;
});
} else {
_activeCount -= 1;
_disposeRecognizerIfInactive();
}
if (mounted && widget.onDragEnd != null) {
widget.onDragEnd!(DraggableDetails(
wasAccepted: wasAccepted,
velocity: velocity,
offset: offset,
));
}
if (wasAccepted && widget.onDragCompleted != null) {
widget.onDragCompleted!();
}
if (!wasAccepted && widget.onDraggableCanceled != null) {
widget.onDraggableCanceled!(velocity, offset);
}
},
);
widget.onDragStarted?.call();
return avatar;
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasOverlay(context));
final bool canDrag = widget.maxSimultaneousDrags == null ||
_activeCount < widget.maxSimultaneousDrags!;
final bool showChild = _activeCount == 0 || widget.childWhenDragging == null;
return Listener(
behavior: widget.hitTestBehavior,
onPointerDown: canDrag ? _routePointer : null,
child: showChild ? widget.child : widget.childWhenDragging,
);
}
}
/// Represents the details when a specific pointer event occurred on
/// the [Draggable].
///
/// This includes the [Velocity] at which the pointer was moving and [Offset]
/// when the draggable event occurred, and whether its [DragTarget] accepted it.
///
/// Also, this is the details object for callbacks that use [DragEndCallback].
class DraggableDetails {
/// Creates details for a [DraggableDetails].
///
/// If [wasAccepted] is not specified, it will default to `false`.
///
/// The [velocity] or [offset] arguments must not be `null`.
DraggableDetails({
this.wasAccepted = false,
required this.velocity,
required this.offset,
});
/// Determines whether the [DragTarget] accepted this draggable.
final bool wasAccepted;
/// The velocity at which the pointer was moving when the specific pointer
/// event occurred on the draggable.
final Velocity velocity;
/// The global position when the specific pointer event occurred on
/// the draggable.
final Offset offset;
}
/// Represents the details when a pointer event occurred on the [DragTarget].
class DragTargetDetails<T> {
/// Creates details for a [DragTarget] callback.
DragTargetDetails({required this.data, required this.offset});
/// The data that was dropped onto this [DragTarget].
final T data;
/// The global position when the specific pointer event occurred on
/// the draggable.
final Offset offset;
}
/// A widget that receives data when a [Draggable] widget is dropped.
///
/// When a draggable is dragged on top of a drag target, the drag target is
/// asked whether it will accept the data the draggable is carrying. If the user
/// does drop the draggable on top of the drag target (and the drag target has
/// indicated that it will accept the draggable's data), then the drag target is
/// asked to accept the draggable's data.
///
/// See also:
///
/// * [Draggable]
/// * [LongPressDraggable]
class DragTarget<T extends Object> extends StatefulWidget {
/// Creates a widget that receives drags.
const DragTarget({
super.key,
required this.builder,
@Deprecated(
'Use onWillAcceptWithDetails instead. '
'This callback is similar to onWillAcceptWithDetails but does not provide drag details. '
'This feature was deprecated after v3.14.0-0.2.pre.'
)
this.onWillAccept,
this.onWillAcceptWithDetails,
@Deprecated(
'Use onAcceptWithDetails instead. '
'This callback is similar to onAcceptWithDetails but does not provide drag details. '
'This feature was deprecated after v3.14.0-0.2.pre.'
)
this.onAccept,
this.onAcceptWithDetails,
this.onLeave,
this.onMove,
this.hitTestBehavior = HitTestBehavior.translucent,
}) : assert(onWillAccept == null || onWillAcceptWithDetails == null, "Don't pass both onWillAccept and onWillAcceptWithDetails.");
/// Called to build the contents of this widget.
///
/// The builder can build different widgets depending on what is being dragged
/// into this drag target.
final DragTargetBuilder<T> builder;
/// Called to determine whether this widget is interested in receiving a given
/// piece of data being dragged over this drag target.
///
/// Called when a piece of data enters the target. This will be followed by
/// either [onAccept] and [onAcceptWithDetails], if the data is dropped, or
/// [onLeave], if the drag leaves the target.
///
/// Equivalent to [onWillAcceptWithDetails], but only includes the data.
///
/// Must not be provided if [onWillAcceptWithDetails] is provided.
@Deprecated(
'Use onWillAcceptWithDetails instead. '
'This callback is similar to onWillAcceptWithDetails but does not provide drag details. '
'This feature was deprecated after v3.14.0-0.2.pre.'
)
final DragTargetWillAccept<T>? onWillAccept;
/// Called to determine whether this widget is interested in receiving a given
/// piece of data being dragged over this drag target.
///
/// Called when a piece of data enters the target. This will be followed by
/// either [onAccept] and [onAcceptWithDetails], if the data is dropped, or
/// [onLeave], if the drag leaves the target.
///
/// Equivalent to [onWillAccept], but with information, including the data,
/// in a [DragTargetDetails].
///
/// Must not be provided if [onWillAccept] is provided.
final DragTargetWillAcceptWithDetails<T>? onWillAcceptWithDetails;
/// Called when an acceptable piece of data was dropped over this drag target.
/// It will not be called if `data` is `null`.
///
/// Equivalent to [onAcceptWithDetails], but only includes the data.
@Deprecated(
'Use onAcceptWithDetails instead. '
'This callback is similar to onAcceptWithDetails but does not provide drag details. '
'This feature was deprecated after v3.14.0-0.2.pre.'
)
final DragTargetAccept<T>? onAccept;
/// Called when an acceptable piece of data was dropped over this drag target.
/// It will not be called if `data` is `null`.
///
/// Equivalent to [onAccept], but with information, including the data, in a
/// [DragTargetDetails].
final DragTargetAcceptWithDetails<T>? onAcceptWithDetails;
/// Called when a given piece of data being dragged over this target leaves
/// the target.
final DragTargetLeave<T>? onLeave;
/// Called when a [Draggable] moves within this [DragTarget]. It will not be
/// called if `data` is `null`.
///
/// This includes entering and leaving the target.
final DragTargetMove<T>? onMove;
/// How to behave during hit testing.
///
/// Defaults to [HitTestBehavior.translucent].
final HitTestBehavior hitTestBehavior;
@override
State<DragTarget<T>> createState() => _DragTargetState<T>();
}
List<T?> _mapAvatarsToData<T extends Object>(List<_DragAvatar<Object>> avatars) {
return avatars.map<T?>((_DragAvatar<Object> avatar) => avatar.data as T?).toList();
}
class _DragTargetState<T extends Object> extends State<DragTarget<T>> {
final List<_DragAvatar<Object>> _candidateAvatars = <_DragAvatar<Object>>[];
final List<_DragAvatar<Object>> _rejectedAvatars = <_DragAvatar<Object>>[];
// On non-web platforms, checks if data Object is equal to type[T] or subtype of [T].
// On web, it does the same, but requires a check for ints and doubles
// because dart doubles and ints are backed by the same kind of object on web.
// JavaScript does not support integers.
bool isExpectedDataType(Object? data, Type type) {
if (kIsWeb && ((type == int && T == double) || (type == double && T == int))) {
return false;
}
return data is T?;
}
bool didEnter(_DragAvatar<Object> avatar) {
assert(!_candidateAvatars.contains(avatar));
assert(!_rejectedAvatars.contains(avatar));
final bool resolvedWillAccept = (widget.onWillAccept == null &&
widget.onWillAcceptWithDetails == null) ||
(widget.onWillAccept != null &&
widget.onWillAccept!(avatar.data as T?)) ||
(widget.onWillAcceptWithDetails != null &&
avatar.data != null &&
widget.onWillAcceptWithDetails!(DragTargetDetails<T>(data: avatar.data! as T, offset: avatar._lastOffset!)));
if (resolvedWillAccept) {
setState(() {
_candidateAvatars.add(avatar);
});
return true;
} else {
setState(() {
_rejectedAvatars.add(avatar);
});
return false;
}
}
void didLeave(_DragAvatar<Object> avatar) {
assert(_candidateAvatars.contains(avatar) || _rejectedAvatars.contains(avatar));
if (!mounted) {
return;
}
setState(() {
_candidateAvatars.remove(avatar);
_rejectedAvatars.remove(avatar);
});
widget.onLeave?.call(avatar.data as T?);
}
void didDrop(_DragAvatar<Object> avatar) {
assert(_candidateAvatars.contains(avatar));
if (!mounted) {
return;
}
setState(() {
_candidateAvatars.remove(avatar);
});
if (avatar.data != null) {
widget.onAccept?.call(avatar.data! as T);
widget.onAcceptWithDetails?.call(DragTargetDetails<T>(data: avatar.data! as T, offset: avatar._lastOffset!));
}
}
void didMove(_DragAvatar<Object> avatar) {
if (!mounted || avatar.data == null) {
return;
}
widget.onMove?.call(DragTargetDetails<T>(data: avatar.data! as T, offset: avatar._lastOffset!));
}
@override
Widget build(BuildContext context) {
return MetaData(
metaData: this,
behavior: widget.hitTestBehavior,
child: widget.builder(context, _mapAvatarsToData<T>(_candidateAvatars), _mapAvatarsToData<Object>(_rejectedAvatars)),
);
}
}
enum _DragEndKind { dropped, canceled }
typedef _OnDragEnd = void Function(Velocity velocity, Offset offset, bool wasAccepted);
// The lifetime of this object is a little dubious right now. Specifically, it
// lives as long as the pointer is down. Arguably it should self-immolate if the
// overlay goes away. _DraggableState has some delicate logic to continue
// needing this object pointer events even after it has been disposed.
class _DragAvatar<T extends Object> extends Drag {
_DragAvatar({
required this.overlayState,
this.data,
this.axis,
required Offset initialPosition,
this.dragStartPoint = Offset.zero,
this.feedback,
this.feedbackOffset = Offset.zero,
this.onDragUpdate,
this.onDragEnd,
required this.ignoringFeedbackSemantics,
required this.ignoringFeedbackPointer,
required this.viewId,
}) : _position = initialPosition {
_entry = OverlayEntry(builder: _build);
overlayState.insert(_entry!);
updateDrag(initialPosition);
}
final T? data;
final Axis? axis;
final Offset dragStartPoint;
final Widget? feedback;
final Offset feedbackOffset;
final DragUpdateCallback? onDragUpdate;
final _OnDragEnd? onDragEnd;
final OverlayState overlayState;
final bool ignoringFeedbackSemantics;
final bool ignoringFeedbackPointer;
final int viewId;
_DragTargetState<Object>? _activeTarget;
final List<_DragTargetState<Object>> _enteredTargets = <_DragTargetState<Object>>[];
Offset _position;
Offset? _lastOffset;
OverlayEntry? _entry;
@override
void update(DragUpdateDetails details) {
final Offset oldPosition = _position;
_position += _restrictAxis(details.delta);
updateDrag(_position);
if (onDragUpdate != null && _position != oldPosition) {
onDragUpdate!(details);
}
}
@override
void end(DragEndDetails details) {
finishDrag(_DragEndKind.dropped, _restrictVelocityAxis(details.velocity));
}
@override
void cancel() {
finishDrag(_DragEndKind.canceled);
}
void updateDrag(Offset globalPosition) {
_lastOffset = globalPosition - dragStartPoint;
_entry!.markNeedsBuild();
final HitTestResult result = HitTestResult();
WidgetsBinding.instance.hitTestInView(result, globalPosition + feedbackOffset, viewId);
final List<_DragTargetState<Object>> targets = _getDragTargets(result.path).toList();
bool listsMatch = false;
if (targets.length >= _enteredTargets.length && _enteredTargets.isNotEmpty) {
listsMatch = true;
final Iterator<_DragTargetState<Object>> iterator = targets.iterator;
for (int i = 0; i < _enteredTargets.length; i += 1) {
iterator.moveNext();
if (iterator.current != _enteredTargets[i]) {
listsMatch = false;
break;
}
}
}
// If everything's the same, report moves, and bail early.
if (listsMatch) {
for (final _DragTargetState<Object> target in _enteredTargets) {
target.didMove(this);
}
return;
}
// Leave old targets.
_leaveAllEntered();
// Enter new targets.
final _DragTargetState<Object>? newTarget = targets.cast<_DragTargetState<Object>?>().firstWhere(
(_DragTargetState<Object>? target) {
if (target == null) {
return false;
}
_enteredTargets.add(target);
return target.didEnter(this);
},
orElse: () => null,
);
// Report moves to the targets.
for (final _DragTargetState<Object> target in _enteredTargets) {
target.didMove(this);
}
_activeTarget = newTarget;
}
Iterable<_DragTargetState<Object>> _getDragTargets(Iterable<HitTestEntry> path) {
// Look for the RenderBoxes that corresponds to the hit target (the hit target
// widgets build RenderMetaData boxes for us for this purpose).
final List<_DragTargetState<Object>> targets = <_DragTargetState<Object>>[];
for (final HitTestEntry entry in path) {
final HitTestTarget target = entry.target;
if (target is RenderMetaData) {
final dynamic metaData = target.metaData;
if (metaData is _DragTargetState && metaData.isExpectedDataType(data, T)) {
targets.add(metaData);
}
}
}
return targets;
}
void _leaveAllEntered() {
for (int i = 0; i < _enteredTargets.length; i += 1) {
_enteredTargets[i].didLeave(this);
}
_enteredTargets.clear();
}
void finishDrag(_DragEndKind endKind, [ Velocity? velocity ]) {
bool wasAccepted = false;
if (endKind == _DragEndKind.dropped && _activeTarget != null) {
_activeTarget!.didDrop(this);
wasAccepted = true;
_enteredTargets.remove(_activeTarget);
}
_leaveAllEntered();
_activeTarget = null;
_entry!.remove();
_entry!.dispose();
_entry = null;
// TODO(ianh): consider passing _entry as well so the client can perform an animation.
onDragEnd?.call(velocity ?? Velocity.zero, _lastOffset!, wasAccepted);
}
Widget _build(BuildContext context) {
final RenderBox box = overlayState.context.findRenderObject()! as RenderBox;
final Offset overlayTopLeft = box.localToGlobal(Offset.zero);
return Positioned(
left: _lastOffset!.dx - overlayTopLeft.dx,
top: _lastOffset!.dy - overlayTopLeft.dy,
child: ExcludeSemantics(
excluding: ignoringFeedbackSemantics,
child: IgnorePointer(
ignoring: ignoringFeedbackPointer,
child: feedback,
),
),
);
}
Velocity _restrictVelocityAxis(Velocity velocity) {
if (axis == null) {
return velocity;
}
return Velocity(
pixelsPerSecond: _restrictAxis(velocity.pixelsPerSecond),
);
}
Offset _restrictAxis(Offset offset) {
if (axis == null) {
return offset;
}
if (axis == Axis.horizontal) {
return Offset(offset.dx, 0.0);
}
return Offset(0.0, offset.dy);
}
}
| flutter/packages/flutter/lib/src/widgets/drag_target.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/drag_target.dart",
"repo_id": "flutter",
"token_count": 11601
} | 671 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui show lerpDouble;
import 'package:flutter/foundation.dart';
import 'package:flutter/painting.dart';
import 'framework.dart' show BuildContext;
/// Defines the size, font variations, color, opacity, and shadows of icons.
///
/// Used by [IconTheme] to control those properties in a widget subtree.
///
/// To obtain the current icon theme, use [IconTheme.of]. To convert an icon
/// theme to a version with all the fields filled in, use
/// [IconThemeData.fallback].
@immutable
class IconThemeData with Diagnosticable {
/// Creates an icon theme data.
///
/// The opacity applies to both explicit and default icon colors. The value
/// is clamped between 0.0 and 1.0.
const IconThemeData({
this.size,
this.fill,
this.weight,
this.grade,
this.opticalSize,
this.color,
double? opacity,
this.shadows,
this.applyTextScaling,
}) : _opacity = opacity,
assert(fill == null || (0.0 <= fill && fill <= 1.0)),
assert(weight == null || (0.0 < weight)),
assert(opticalSize == null || (0.0 < opticalSize));
/// Creates an icon theme with some reasonable default values.
///
/// The [size] is 24.0, [fill] is 0.0, [weight] is 400.0, [grade] is 0.0,
/// opticalSize is 48.0, [color] is black, and [opacity] is 1.0.
const IconThemeData.fallback()
: size = 24.0,
fill = 0.0,
weight = 400.0,
grade = 0.0,
opticalSize = 48.0,
color = const Color(0xFF000000),
_opacity = 1.0,
shadows = null,
applyTextScaling = false;
/// Creates a copy of this icon theme but with the given fields replaced with
/// the new values.
IconThemeData copyWith({
double? size,
double? fill,
double? weight,
double? grade,
double? opticalSize,
Color? color,
double? opacity,
List<Shadow>? shadows,
bool? applyTextScaling,
}) {
return IconThemeData(
size: size ?? this.size,
fill: fill ?? this.fill,
weight: weight ?? this.weight,
grade: grade ?? this.grade,
opticalSize: opticalSize ?? this.opticalSize,
color: color ?? this.color,
opacity: opacity ?? this.opacity,
shadows: shadows ?? this.shadows,
applyTextScaling: applyTextScaling ?? this.applyTextScaling,
);
}
/// Returns a new icon theme that matches this icon theme but with some values
/// replaced by the non-null parameters of the given icon theme. If the given
/// icon theme is null, returns this icon theme.
IconThemeData merge(IconThemeData? other) {
if (other == null) {
return this;
}
return copyWith(
size: other.size,
fill: other.fill,
weight: other.weight,
grade: other.grade,
opticalSize: other.opticalSize,
color: other.color,
opacity: other.opacity,
shadows: other.shadows,
applyTextScaling: other.applyTextScaling,
);
}
/// Called by [IconTheme.of] to convert this instance to an [IconThemeData]
/// that fits the given [BuildContext].
///
/// This method gives the ambient [IconThemeData] a chance to update itself,
/// after it's been retrieved by [IconTheme.of], and before being returned as
/// the final result. For instance, [CupertinoIconThemeData] overrides this method
/// to resolve [color], in case [color] is a [CupertinoDynamicColor] and needs
/// to be resolved against the given [BuildContext] before it can be used as a
/// regular [Color].
///
/// The default implementation returns this [IconThemeData] as-is.
///
/// See also:
///
/// * [CupertinoIconThemeData.resolve] an implementation that resolves
/// the color of [CupertinoIconThemeData] before returning.
IconThemeData resolve(BuildContext context) => this;
/// Whether all the properties (except shadows) of this object are non-null.
bool get isConcrete => size != null
&& fill != null
&& weight != null
&& grade != null
&& opticalSize != null
&& color != null
&& opacity != null
&& applyTextScaling != null;
/// The default for [Icon.size].
///
/// Falls back to 24.0.
final double? size;
/// The default for [Icon.fill].
///
/// Falls back to 0.0.
final double? fill;
/// The default for [Icon.weight].
///
/// Falls back to 400.0.
final double? weight;
/// The default for [Icon.grade].
///
/// Falls back to 0.0.
final double? grade;
/// The default for [Icon.opticalSize].
///
/// Falls back to 48.0.
final double? opticalSize;
/// The default for [Icon.color].
///
/// In material apps, if there is a [Theme] without any [IconTheme]s
/// specified, icon colors default to white if [ThemeData.brightness] is dark
/// and black if [ThemeData.brightness] is light.
///
/// Otherwise, falls back to black.
final Color? color;
/// An opacity to apply to both explicit and default icon colors.
///
/// Falls back to 1.0.
double? get opacity => _opacity == null ? null : clampDouble(_opacity, 0.0, 1.0);
final double? _opacity;
/// The default for [Icon.shadows].
final List<Shadow>? shadows;
/// The default for [Icon.applyTextScaling].
final bool? applyTextScaling;
/// Linearly interpolate between two icon theme data objects.
///
/// {@macro dart.ui.shadow.lerp}
static IconThemeData lerp(IconThemeData? a, IconThemeData? b, double t) {
if (identical(a, b) && a != null) {
return a;
}
return IconThemeData(
size: ui.lerpDouble(a?.size, b?.size, t),
fill: ui.lerpDouble(a?.fill, b?.fill, t),
weight: ui.lerpDouble(a?.weight, b?.weight, t),
grade: ui.lerpDouble(a?.grade, b?.grade, t),
opticalSize: ui.lerpDouble(a?.opticalSize, b?.opticalSize, t),
color: Color.lerp(a?.color, b?.color, t),
opacity: ui.lerpDouble(a?.opacity, b?.opacity, t),
shadows: Shadow.lerpList(a?.shadows, b?.shadows, t),
applyTextScaling: t < 0.5 ? a?.applyTextScaling : b?.applyTextScaling,
);
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is IconThemeData
&& other.size == size
&& other.fill == fill
&& other.weight == weight
&& other.grade == grade
&& other.opticalSize == opticalSize
&& other.color == color
&& other.opacity == opacity
&& listEquals(other.shadows, shadows)
&& other.applyTextScaling == applyTextScaling;
}
@override
int get hashCode => Object.hash(
size,
fill,
weight,
grade,
opticalSize,
color,
opacity,
shadows == null ? null : Object.hashAll(shadows!),
applyTextScaling,
);
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(DoubleProperty('size', size, defaultValue: null));
properties.add(DoubleProperty('fill', fill, defaultValue: null));
properties.add(DoubleProperty('weight', weight, defaultValue: null));
properties.add(DoubleProperty('grade', grade, defaultValue: null));
properties.add(DoubleProperty('opticalSize', opticalSize, defaultValue: null));
properties.add(ColorProperty('color', color, defaultValue: null));
properties.add(DoubleProperty('opacity', opacity, defaultValue: null));
properties.add(IterableProperty<Shadow>('shadows', shadows, defaultValue: null));
properties.add(DiagnosticsProperty<bool>('applyTextScaling', applyTextScaling, defaultValue: null));
}
}
| flutter/packages/flutter/lib/src/widgets/icon_theme_data.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/icon_theme_data.dart",
"repo_id": "flutter",
"token_count": 2704
} | 672 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'basic.dart';
import 'debug.dart';
import 'framework.dart';
import 'gesture_detector.dart';
import 'navigator.dart';
import 'transitions.dart';
/// A widget that modifies the size of the [SemanticsNode.rect] created by its
/// child widget.
///
/// It clips the focus in potentially four directions based on the
/// specified [EdgeInsets].
///
/// The size of the accessibility focus is adjusted based on value changes
/// inside the given [ValueNotifier].
///
/// See also:
///
/// * [ModalBarrier], which utilizes this widget to adjust the barrier focus
/// size based on the size of the content layer rendered on top of it.
class _SemanticsClipper extends SingleChildRenderObjectWidget{
/// creates a [SemanticsClipper] that updates the size of the
/// [SemanticsNode.rect] of its child based on the value inside the provided
/// [ValueNotifier], or a default value of [EdgeInsets.zero].
const _SemanticsClipper({
super.child,
required this.clipDetailsNotifier,
});
/// The [ValueNotifier] whose value determines how the child's
/// [SemanticsNode.rect] should be clipped in four directions.
final ValueNotifier<EdgeInsets> clipDetailsNotifier;
@override
_RenderSemanticsClipper createRenderObject(BuildContext context) {
return _RenderSemanticsClipper(clipDetailsNotifier: clipDetailsNotifier,);
}
@override
void updateRenderObject(BuildContext context, _RenderSemanticsClipper renderObject) {
renderObject.clipDetailsNotifier = clipDetailsNotifier;
}
}
/// Updates the [SemanticsNode.rect] of its child based on the value inside
/// provided [ValueNotifier].
class _RenderSemanticsClipper extends RenderProxyBox {
/// Creates a [RenderProxyBox] that Updates the [SemanticsNode.rect] of its child
/// based on the value inside provided [ValueNotifier].
_RenderSemanticsClipper({
required ValueNotifier<EdgeInsets> clipDetailsNotifier,
RenderBox? child,
}) : _clipDetailsNotifier = clipDetailsNotifier,
super(child);
ValueNotifier<EdgeInsets> _clipDetailsNotifier;
/// The getter and setter retrieves / updates the [ValueNotifier] associated
/// with this clipper.
ValueNotifier<EdgeInsets> get clipDetailsNotifier => _clipDetailsNotifier;
set clipDetailsNotifier (ValueNotifier<EdgeInsets> newNotifier) {
if (_clipDetailsNotifier == newNotifier) {
return;
}
if (attached) {
_clipDetailsNotifier.removeListener(markNeedsSemanticsUpdate);
}
_clipDetailsNotifier = newNotifier;
_clipDetailsNotifier.addListener(markNeedsSemanticsUpdate);
markNeedsSemanticsUpdate();
}
@override
Rect get semanticBounds {
final EdgeInsets clipDetails = _clipDetailsNotifier.value;
final Rect originalRect = super.semanticBounds;
final Rect clippedRect = Rect.fromLTRB(
originalRect.left + clipDetails.left,
originalRect.top + clipDetails.top,
originalRect.right - clipDetails.right,
originalRect.bottom - clipDetails.bottom,
);
return clippedRect;
}
@override
void attach(PipelineOwner owner) {
super.attach(owner);
clipDetailsNotifier.addListener(markNeedsSemanticsUpdate);
}
@override
void detach() {
clipDetailsNotifier.removeListener(markNeedsSemanticsUpdate);
super.detach();
}
@override
void describeSemanticsConfiguration(SemanticsConfiguration config) {
super.describeSemanticsConfiguration(config);
config.isSemanticBoundary = true;
}
}
/// A widget that prevents the user from interacting with widgets behind itself.
///
/// The modal barrier is the scrim that is rendered behind each route, which
/// generally prevents the user from interacting with the route below the
/// current route, and normally partially obscures such routes.
///
/// For example, when a dialog is on the screen, the page below the dialog is
/// usually darkened by the modal barrier.
///
/// See also:
///
/// * [ModalRoute], which indirectly uses this widget.
/// * [AnimatedModalBarrier], which is similar but takes an animated [color]
/// instead of a single color value.
class ModalBarrier extends StatelessWidget {
/// Creates a widget that blocks user interaction.
const ModalBarrier({
super.key,
this.color,
this.dismissible = true,
this.onDismiss,
this.semanticsLabel,
this.barrierSemanticsDismissible = true,
this.clipDetailsNotifier,
this.semanticsOnTapHint,
});
/// If non-null, fill the barrier with this color.
///
/// See also:
///
/// * [ModalRoute.barrierColor], which controls this property for the
/// [ModalBarrier] built by [ModalRoute] pages.
final Color? color;
/// Specifies if the barrier will be dismissed when the user taps on it.
///
/// If true, and [onDismiss] is non-null, [onDismiss] will be called,
/// otherwise the current route will be popped from the ambient [Navigator].
///
/// If false, tapping on the barrier will do nothing.
///
/// See also:
///
/// * [ModalRoute.barrierDismissible], which controls this property for the
/// [ModalBarrier] built by [ModalRoute] pages.
final bool dismissible;
/// {@template flutter.widgets.ModalBarrier.onDismiss}
/// Called when the barrier is being dismissed.
///
/// If non-null [onDismiss] will be called in place of popping the current
/// route. It is up to the callback to handle dismissing the barrier.
///
/// If null, the ambient [Navigator]'s current route will be popped.
///
/// This field is ignored if [dismissible] is false.
/// {@endtemplate}
final VoidCallback? onDismiss;
/// Whether the modal barrier semantics are included in the semantics tree.
///
/// See also:
///
/// * [ModalRoute.semanticsDismissible], which controls this property for
/// the [ModalBarrier] built by [ModalRoute] pages.
final bool? barrierSemanticsDismissible;
/// Semantics label used for the barrier if it is [dismissible].
///
/// The semantics label is read out by accessibility tools (e.g. TalkBack
/// on Android and VoiceOver on iOS) when the barrier is focused.
///
/// See also:
///
/// * [ModalRoute.barrierLabel], which controls this property for the
/// [ModalBarrier] built by [ModalRoute] pages.
final String? semanticsLabel;
/// {@template flutter.widgets.ModalBarrier.clipDetailsNotifier}
/// Contains a value of type [EdgeInsets] that specifies how the
/// [SemanticsNode.rect] of the widget should be clipped.
///
/// See also:
///
/// * [_SemanticsClipper], which utilizes the value inside to update the
/// [SemanticsNode.rect] for its child.
/// {@endtemplate}
final ValueNotifier<EdgeInsets>? clipDetailsNotifier;
/// {@macro flutter.material.ModalBottomSheetRoute.barrierOnTapHint}
final String? semanticsOnTapHint;
@override
Widget build(BuildContext context) {
assert(!dismissible || semanticsLabel == null || debugCheckHasDirectionality(context));
final bool platformSupportsDismissingBarrier;
switch (defaultTargetPlatform) {
case TargetPlatform.fuchsia:
case TargetPlatform.linux:
case TargetPlatform.windows:
platformSupportsDismissingBarrier = false;
case TargetPlatform.android:
case TargetPlatform.iOS:
case TargetPlatform.macOS:
platformSupportsDismissingBarrier = true;
}
final bool semanticsDismissible = dismissible && platformSupportsDismissingBarrier;
final bool modalBarrierSemanticsDismissible = barrierSemanticsDismissible ?? semanticsDismissible;
void handleDismiss() {
if (dismissible) {
if (onDismiss != null) {
onDismiss!();
} else {
Navigator.maybePop(context);
}
} else {
SystemSound.play(SystemSoundType.alert);
}
}
Widget barrier = Semantics(
onTapHint: semanticsOnTapHint,
onTap: semanticsDismissible && semanticsLabel != null ? handleDismiss : null,
onDismiss: semanticsDismissible && semanticsLabel != null ? handleDismiss : null,
label: semanticsDismissible ? semanticsLabel : null,
textDirection: semanticsDismissible && semanticsLabel != null ? Directionality.of(context) : null,
child: MouseRegion(
cursor: SystemMouseCursors.basic,
child: ConstrainedBox(
constraints: const BoxConstraints.expand(),
child: color == null ? null : ColoredBox(
color: color!,
),
),
),
);
// Developers can set [dismissible: true] and [barrierSemanticsDismissible: true]
// to allow assistive technology users to dismiss a modal BottomSheet by
// tapping on the Scrim focus.
// On iOS, some modal barriers are not dismissible in accessibility mode.
final bool excluding = !semanticsDismissible || !modalBarrierSemanticsDismissible;
if (!excluding && clipDetailsNotifier != null) {
barrier = _SemanticsClipper(
clipDetailsNotifier: clipDetailsNotifier!,
child: barrier,
);
}
return BlockSemantics(
child: ExcludeSemantics(
excluding: excluding,
child: _ModalBarrierGestureDetector(
onDismiss: handleDismiss,
child: barrier,
),
),
);
}
}
/// A widget that prevents the user from interacting with widgets behind itself,
/// and can be configured with an animated color value.
///
/// The modal barrier is the scrim that is rendered behind each route, which
/// generally prevents the user from interacting with the route below the
/// current route, and normally partially obscures such routes.
///
/// For example, when a dialog is on the screen, the page below the dialog is
/// usually darkened by the modal barrier.
///
/// This widget is similar to [ModalBarrier] except that it takes an animated
/// [color] instead of a single color.
///
/// See also:
///
/// * [ModalRoute], which uses this widget.
class AnimatedModalBarrier extends AnimatedWidget {
/// Creates a widget that blocks user interaction.
const AnimatedModalBarrier({
super.key,
required Animation<Color?> color,
this.dismissible = true,
this.semanticsLabel,
this.barrierSemanticsDismissible,
this.onDismiss,
this.clipDetailsNotifier,
this.semanticsOnTapHint,
}) : super(listenable: color);
/// If non-null, fill the barrier with this color.
///
/// See also:
///
/// * [ModalRoute.barrierColor], which controls this property for the
/// [AnimatedModalBarrier] built by [ModalRoute] pages.
Animation<Color?> get color => listenable as Animation<Color?>;
/// Whether touching the barrier will pop the current route off the [Navigator].
///
/// See also:
///
/// * [ModalRoute.barrierDismissible], which controls this property for the
/// [AnimatedModalBarrier] built by [ModalRoute] pages.
final bool dismissible;
/// Semantics label used for the barrier if it is [dismissible].
///
/// The semantics label is read out by accessibility tools (e.g. TalkBack
/// on Android and VoiceOver on iOS) when the barrier is focused.
/// See also:
///
/// * [ModalRoute.barrierLabel], which controls this property for the
/// [ModalBarrier] built by [ModalRoute] pages.
final String? semanticsLabel;
/// Whether the modal barrier semantics are included in the semantics tree.
///
/// See also:
///
/// * [ModalRoute.semanticsDismissible], which controls this property for
/// the [ModalBarrier] built by [ModalRoute] pages.
final bool? barrierSemanticsDismissible;
/// {@macro flutter.widgets.ModalBarrier.onDismiss}
final VoidCallback? onDismiss;
/// {@macro flutter.widgets.ModalBarrier.clipDetailsNotifier}
final ValueNotifier<EdgeInsets>? clipDetailsNotifier;
/// This hint text instructs users what they are able to do when they tap on
/// the [ModalBarrier]
///
/// E.g. If the hint text is 'close bottom sheet", it will be announced as
/// "Double tap to close bottom sheet".
///
/// If this value is null, the default onTapHint will be applied, resulting
/// in the announcement of 'Double tap to activate'.
final String? semanticsOnTapHint;
@override
Widget build(BuildContext context) {
return ModalBarrier(
color: color.value,
dismissible: dismissible,
semanticsLabel: semanticsLabel,
barrierSemanticsDismissible: barrierSemanticsDismissible,
onDismiss: onDismiss,
clipDetailsNotifier: clipDetailsNotifier,
semanticsOnTapHint: semanticsOnTapHint,
);
}
}
// Recognizes tap down by any pointer button.
//
// It is similar to [TapGestureRecognizer.onTapDown], but accepts any single
// button, which means the gesture also takes parts in gesture arenas.
class _AnyTapGestureRecognizer extends BaseTapGestureRecognizer {
_AnyTapGestureRecognizer();
VoidCallback? onAnyTapUp;
@protected
@override
bool isPointerAllowed(PointerDownEvent event) {
if (onAnyTapUp == null) {
return false;
}
return super.isPointerAllowed(event);
}
@protected
@override
void handleTapDown({PointerDownEvent? down}) {
// Do nothing.
}
@protected
@override
void handleTapUp({PointerDownEvent? down, PointerUpEvent? up}) {
if (onAnyTapUp != null) {
invokeCallback('onAnyTapUp', onAnyTapUp!);
}
}
@protected
@override
void handleTapCancel({PointerDownEvent? down, PointerCancelEvent? cancel, String? reason}) {
// Do nothing.
}
@override
String get debugDescription => 'any tap';
}
class _AnyTapGestureRecognizerFactory extends GestureRecognizerFactory<_AnyTapGestureRecognizer> {
const _AnyTapGestureRecognizerFactory({this.onAnyTapUp});
final VoidCallback? onAnyTapUp;
@override
_AnyTapGestureRecognizer constructor() => _AnyTapGestureRecognizer();
@override
void initializer(_AnyTapGestureRecognizer instance) {
instance.onAnyTapUp = onAnyTapUp;
}
}
// A GestureDetector used by ModalBarrier. It only has one callback,
// [onAnyTapDown], which recognizes tap down unconditionally.
class _ModalBarrierGestureDetector extends StatelessWidget {
const _ModalBarrierGestureDetector({
required this.child,
required this.onDismiss,
});
/// The widget below this widget in the tree.
/// See [RawGestureDetector.child].
final Widget child;
/// Immediately called when an event that should dismiss the modal barrier
/// has happened.
final VoidCallback onDismiss;
@override
Widget build(BuildContext context) {
final Map<Type, GestureRecognizerFactory> gestures = <Type, GestureRecognizerFactory>{
_AnyTapGestureRecognizer: _AnyTapGestureRecognizerFactory(onAnyTapUp: onDismiss),
};
return RawGestureDetector(
gestures: gestures,
behavior: HitTestBehavior.opaque,
child: child,
);
}
}
| flutter/packages/flutter/lib/src/widgets/modal_barrier.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/modal_barrier.dart",
"repo_id": "flutter",
"token_count": 4862
} | 673 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/animation.dart';
import 'package:flutter/foundation.dart';
import 'scroll_context.dart';
import 'scroll_physics.dart';
import 'scroll_position.dart';
import 'scroll_position_with_single_context.dart';
// Examples can assume:
// TrackingScrollController _trackingScrollController = TrackingScrollController();
/// Signature for when a [ScrollController] has added or removed a
/// [ScrollPosition].
///
/// Since a [ScrollPosition] is not created and attached to a controller until
/// the [Scrollable] is built, this can be used to respond to the position being
/// attached to a controller.
///
/// By having access to the position directly, additional listeners can be
/// applied to aspects of the scroll position, like
/// [ScrollPosition.isScrollingNotifier].
///
/// Used by [ScrollController.onAttach] and [ScrollController.onDetach].
typedef ScrollControllerCallback = void Function(ScrollPosition position);
/// Controls a scrollable widget.
///
/// Scroll controllers are typically stored as member variables in [State]
/// objects and are reused in each [State.build]. A single scroll controller can
/// be used to control multiple scrollable widgets, but some operations, such
/// as reading the scroll [offset], require the controller to be used with a
/// single scrollable widget.
///
/// A scroll controller creates a [ScrollPosition] to manage the state specific
/// to an individual [Scrollable] widget. To use a custom [ScrollPosition],
/// subclass [ScrollController] and override [createScrollPosition].
///
/// {@macro flutter.widgets.scrollPosition.listening}
///
/// Typically used with [ListView], [GridView], [CustomScrollView].
///
/// See also:
///
/// * [ListView], [GridView], [CustomScrollView], which can be controlled by a
/// [ScrollController].
/// * [Scrollable], which is the lower-level widget that creates and associates
/// [ScrollPosition] objects with [ScrollController] objects.
/// * [PageController], which is an analogous object for controlling a
/// [PageView].
/// * [ScrollPosition], which manages the scroll offset for an individual
/// scrolling widget.
/// * [ScrollNotification] and [NotificationListener], which can be used to
/// listen to scrolling occur without using a [ScrollController].
class ScrollController extends ChangeNotifier {
/// Creates a controller for a scrollable widget.
ScrollController({
double initialScrollOffset = 0.0,
this.keepScrollOffset = true,
this.debugLabel,
this.onAttach,
this.onDetach,
}) : _initialScrollOffset = initialScrollOffset {
if (kFlutterMemoryAllocationsEnabled) {
ChangeNotifier.maybeDispatchObjectCreation(this);
}
}
/// The initial value to use for [offset].
///
/// New [ScrollPosition] objects that are created and attached to this
/// controller will have their offset initialized to this value
/// if [keepScrollOffset] is false or a scroll offset hasn't been saved yet.
///
/// Defaults to 0.0.
double get initialScrollOffset => _initialScrollOffset;
final double _initialScrollOffset;
/// Each time a scroll completes, save the current scroll [offset] with
/// [PageStorage] and restore it if this controller's scrollable is recreated.
///
/// If this property is set to false, the scroll offset is never saved
/// and [initialScrollOffset] is always used to initialize the scroll
/// offset. If true (the default), the initial scroll offset is used the
/// first time the controller's scrollable is created, since there's no
/// scroll offset to restore yet. Subsequently the saved offset is
/// restored and [initialScrollOffset] is ignored.
///
/// See also:
///
/// * [PageStorageKey], which should be used when more than one
/// scrollable appears in the same route, to distinguish the [PageStorage]
/// locations used to save scroll offsets.
final bool keepScrollOffset;
/// Called when a [ScrollPosition] is attached to the scroll controller.
///
/// Since a scroll position is not attached until a [Scrollable] is actually
/// built, this can be used to respond to a new position being attached.
///
/// At the time that a scroll position is attached, the [ScrollMetrics], such as
/// the [ScrollMetrics.maxScrollExtent], are not yet available. These are not
/// determined until the [Scrollable] has finished laying out its contents and
/// computing things like the full extent of that content.
/// [ScrollPosition.hasContentDimensions] can be used to know when the
/// metrics are available, or a [ScrollMetricsNotification] can be used,
/// discussed further below.
///
/// {@tool dartpad}
/// This sample shows how to apply a listener to the
/// [ScrollPosition.isScrollingNotifier] using [ScrollController.onAttach].
/// This is used to change the [AppBar]'s color when scrolling is occurring.
///
/// ** See code in examples/api/lib/widgets/scroll_position/scroll_controller_on_attach.0.dart **
/// {@end-tool}
final ScrollControllerCallback? onAttach;
/// Called when a [ScrollPosition] is detached from the scroll controller.
///
/// {@tool dartpad}
/// This sample shows how to apply a listener to the
/// [ScrollPosition.isScrollingNotifier] using [ScrollController.onAttach]
/// & [ScrollController.onDetach].
/// This is used to change the [AppBar]'s color when scrolling is occurring.
///
/// ** See code in examples/api/lib/widgets/scroll_position/scroll_controller_on_attach.0.dart **
/// {@end-tool}
final ScrollControllerCallback? onDetach;
/// A label that is used in the [toString] output. Intended to aid with
/// identifying scroll controller instances in debug output.
final String? debugLabel;
/// The currently attached positions.
///
/// This should not be mutated directly. [ScrollPosition] objects can be added
/// and removed using [attach] and [detach].
Iterable<ScrollPosition> get positions => _positions;
final List<ScrollPosition> _positions = <ScrollPosition>[];
/// Whether any [ScrollPosition] objects have attached themselves to the
/// [ScrollController] using the [attach] method.
///
/// If this is false, then members that interact with the [ScrollPosition],
/// such as [position], [offset], [animateTo], and [jumpTo], must not be
/// called.
bool get hasClients => _positions.isNotEmpty;
/// Returns the attached [ScrollPosition], from which the actual scroll offset
/// of the [ScrollView] can be obtained.
///
/// Calling this is only valid when only a single position is attached.
ScrollPosition get position {
assert(_positions.isNotEmpty, 'ScrollController not attached to any scroll views.');
assert(_positions.length == 1, 'ScrollController attached to multiple scroll views.');
return _positions.single;
}
/// The current scroll offset of the scrollable widget.
///
/// Requires the controller to be controlling exactly one scrollable widget.
double get offset => position.pixels;
/// Animates the position from its current value to the given value.
///
/// Any active animation is canceled. If the user is currently scrolling, that
/// action is canceled.
///
/// The returned [Future] will complete when the animation ends, whether it
/// completed successfully or whether it was interrupted prematurely.
///
/// An animation will be interrupted whenever the user attempts to scroll
/// manually, or whenever another activity is started, or whenever the
/// animation reaches the edge of the viewport and attempts to overscroll. (If
/// the [ScrollPosition] does not overscroll but instead allows scrolling
/// beyond the extents, then going beyond the extents will not interrupt the
/// animation.)
///
/// The animation is indifferent to changes to the viewport or content
/// dimensions.
///
/// Once the animation has completed, the scroll position will attempt to
/// begin a ballistic activity in case its value is not stable (for example,
/// if it is scrolled beyond the extents and in that situation the scroll
/// position would normally bounce back).
///
/// The duration must not be zero. To jump to a particular value without an
/// animation, use [jumpTo].
///
/// When calling [animateTo] in widget tests, `await`ing the returned
/// [Future] may cause the test to hang and timeout. Instead, use
/// [WidgetTester.pumpAndSettle].
Future<void> animateTo(
double offset, {
required Duration duration,
required Curve curve,
}) async {
assert(_positions.isNotEmpty, 'ScrollController not attached to any scroll views.');
await Future.wait<void>(<Future<void>>[
for (int i = 0; i < _positions.length; i += 1) _positions[i].animateTo(offset, duration: duration, curve: curve),
]);
}
/// Jumps the scroll position from its current value to the given value,
/// without animation, and without checking if the new value is in range.
///
/// Any active animation is canceled. If the user is currently scrolling, that
/// action is canceled.
///
/// If this method changes the scroll position, a sequence of start/update/end
/// scroll notifications will be dispatched. No overscroll notifications can
/// be generated by this method.
///
/// Immediately after the jump, a ballistic activity is started, in case the
/// value was out of range.
void jumpTo(double value) {
assert(_positions.isNotEmpty, 'ScrollController not attached to any scroll views.');
for (final ScrollPosition position in List<ScrollPosition>.of(_positions)) {
position.jumpTo(value);
}
}
/// Register the given position with this controller.
///
/// After this function returns, the [animateTo] and [jumpTo] methods on this
/// controller will manipulate the given position.
void attach(ScrollPosition position) {
assert(!_positions.contains(position));
_positions.add(position);
position.addListener(notifyListeners);
if (onAttach != null) {
onAttach!(position);
}
}
/// Unregister the given position with this controller.
///
/// After this function returns, the [animateTo] and [jumpTo] methods on this
/// controller will not manipulate the given position.
void detach(ScrollPosition position) {
assert(_positions.contains(position));
if (onDetach != null) {
onDetach!(position);
}
position.removeListener(notifyListeners);
_positions.remove(position);
}
@override
void dispose() {
for (final ScrollPosition position in _positions) {
position.removeListener(notifyListeners);
}
super.dispose();
}
/// Creates a [ScrollPosition] for use by a [Scrollable] widget.
///
/// Subclasses can override this function to customize the [ScrollPosition]
/// used by the scrollable widgets they control. For example, [PageController]
/// overrides this function to return a page-oriented scroll position
/// subclass that keeps the same page visible when the scrollable widget
/// resizes.
///
/// By default, returns a [ScrollPositionWithSingleContext].
///
/// The arguments are generally passed to the [ScrollPosition] being created:
///
/// * `physics`: An instance of [ScrollPhysics] that determines how the
/// [ScrollPosition] should react to user interactions, how it should
/// simulate scrolling when released or flung, etc. The value will not be
/// null. It typically comes from the [ScrollView] or other widget that
/// creates the [Scrollable], or, if none was provided, from the ambient
/// [ScrollConfiguration].
/// * `context`: A [ScrollContext] used for communicating with the object
/// that is to own the [ScrollPosition] (typically, this is the
/// [Scrollable] itself).
/// * `oldPosition`: If this is not the first time a [ScrollPosition] has
/// been created for this [Scrollable], this will be the previous instance.
/// This is used when the environment has changed and the [Scrollable]
/// needs to recreate the [ScrollPosition] object. It is null the first
/// time the [ScrollPosition] is created.
ScrollPosition createScrollPosition(
ScrollPhysics physics,
ScrollContext context,
ScrollPosition? oldPosition,
) {
return ScrollPositionWithSingleContext(
physics: physics,
context: context,
initialPixels: initialScrollOffset,
keepScrollOffset: keepScrollOffset,
oldPosition: oldPosition,
debugLabel: debugLabel,
);
}
@override
String toString() {
final List<String> description = <String>[];
debugFillDescription(description);
return '${describeIdentity(this)}(${description.join(", ")})';
}
/// Add additional information to the given description for use by [toString].
///
/// This method makes it easier for subclasses to coordinate to provide a
/// high-quality [toString] implementation. The [toString] implementation on
/// the [ScrollController] base class calls [debugFillDescription] to collect
/// useful information from subclasses to incorporate into its return value.
///
/// Implementations of this method should start with a call to the inherited
/// method, as in `super.debugFillDescription(description)`.
@mustCallSuper
void debugFillDescription(List<String> description) {
if (debugLabel != null) {
description.add(debugLabel!);
}
if (initialScrollOffset != 0.0) {
description.add('initialScrollOffset: ${initialScrollOffset.toStringAsFixed(1)}, ');
}
if (_positions.isEmpty) {
description.add('no clients');
} else if (_positions.length == 1) {
// Don't actually list the client itself, since its toString may refer to us.
description.add('one client, offset ${offset.toStringAsFixed(1)}');
} else {
description.add('${_positions.length} clients');
}
}
}
// Examples can assume:
// TrackingScrollController? _trackingScrollController;
/// A [ScrollController] whose [initialScrollOffset] tracks its most recently
/// updated [ScrollPosition].
///
/// This class can be used to synchronize the scroll offset of two or more
/// lazily created scroll views that share a single [TrackingScrollController].
/// It tracks the most recently updated scroll position and reports it as its
/// `initialScrollOffset`.
///
/// {@tool snippet}
///
/// In this example each [PageView] page contains a [ListView] and all three
/// [ListView]'s share a [TrackingScrollController]. The scroll offsets of all
/// three list views will track each other, to the extent that's possible given
/// the different list lengths.
///
/// ```dart
/// PageView(
/// children: <Widget>[
/// ListView(
/// controller: _trackingScrollController,
/// children: List<Widget>.generate(100, (int i) => Text('page 0 item $i')).toList(),
/// ),
/// ListView(
/// controller: _trackingScrollController,
/// children: List<Widget>.generate(200, (int i) => Text('page 1 item $i')).toList(),
/// ),
/// ListView(
/// controller: _trackingScrollController,
/// children: List<Widget>.generate(300, (int i) => Text('page 2 item $i')).toList(),
/// ),
/// ],
/// )
/// ```
/// {@end-tool}
///
/// In this example the `_trackingController` would have been created by the
/// stateful widget that built the widget tree.
class TrackingScrollController extends ScrollController {
/// Creates a scroll controller that continually updates its
/// [initialScrollOffset] to match the last scroll notification it received.
TrackingScrollController({
super.initialScrollOffset,
super.keepScrollOffset,
super.debugLabel,
super.onAttach,
super.onDetach,
});
final Map<ScrollPosition, VoidCallback> _positionToListener = <ScrollPosition, VoidCallback>{};
ScrollPosition? _lastUpdated;
double? _lastUpdatedOffset;
/// The last [ScrollPosition] to change. Returns null if there aren't any
/// attached scroll positions, or there hasn't been any scrolling yet, or the
/// last [ScrollPosition] to change has since been removed.
ScrollPosition? get mostRecentlyUpdatedPosition => _lastUpdated;
/// Returns the scroll offset of the [mostRecentlyUpdatedPosition] or, if that
/// is null, the initial scroll offset provided to the constructor.
///
/// See also:
///
/// * [ScrollController.initialScrollOffset], which this overrides.
@override
double get initialScrollOffset => _lastUpdatedOffset ?? super.initialScrollOffset;
@override
void attach(ScrollPosition position) {
super.attach(position);
assert(!_positionToListener.containsKey(position));
_positionToListener[position] = () {
_lastUpdated = position;
_lastUpdatedOffset = position.pixels;
};
position.addListener(_positionToListener[position]!);
}
@override
void detach(ScrollPosition position) {
super.detach(position);
assert(_positionToListener.containsKey(position));
position.removeListener(_positionToListener[position]!);
_positionToListener.remove(position);
if (_lastUpdated == position) {
_lastUpdated = null;
}
if (_positionToListener.isEmpty) {
_lastUpdatedOffset = null;
}
}
@override
void dispose() {
for (final ScrollPosition position in positions) {
assert(_positionToListener.containsKey(position));
position.removeListener(_positionToListener[position]!);
}
super.dispose();
}
}
| flutter/packages/flutter/lib/src/widgets/scroll_controller.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/scroll_controller.dart",
"repo_id": "flutter",
"token_count": 4892
} | 674 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
/// Service extension constants for the widgets library.
///
/// These constants will be used when registering service extensions in the
/// framework, and they will also be used by tools and services that call these
/// service extensions.
///
/// The String value for each of these extension names should be accessed by
/// calling the `.name` property on the enum value.
enum WidgetsServiceExtensions {
/// Name of service extension that, when called, will output a string
/// representation of this app's widget tree to console.
///
/// See also:
///
/// * [WidgetsBinding.initServiceExtensions], where the service extension is
/// registered.
debugDumpApp,
/// Name of service extension that, when called, will output a string
/// representation of the focus tree to the console.
///
/// See also:
///
/// * [WidgetsBinding.initServiceExtensions], where the service extension is
/// registered.
debugDumpFocusTree,
/// Name of service extension that, when called, will overlay a performance
/// graph on top of this app.
///
/// See also:
///
/// * [WidgetsApp.showPerformanceOverlayOverride], which is the flag
/// that this service extension exposes.
/// * [WidgetsBinding.initServiceExtensions], where the service extension is
/// registered.
showPerformanceOverlay,
/// Name of service extension that, when called, will return whether the first
/// 'Flutter.Frame' event has been reported on the Extension stream.
///
/// See also:
///
/// * [WidgetsBinding.initServiceExtensions], where the service extension is
/// registered.
didSendFirstFrameEvent,
/// Name of service extension that, when called, will return whether the first
/// frame has been rasterized and the trace event 'Rasterized first useful
/// frame' has been sent out.
///
/// See also:
///
/// * [WidgetsBinding.initServiceExtensions], where the service extension is
/// registered.
didSendFirstFrameRasterizedEvent,
/// Name of service extension that, when called, will reassemble the
/// application.
///
/// See also:
///
/// * [WidgetsBinding.initServiceExtensions], where the service extension is
/// registered.
fastReassemble,
/// Name of service extension that, when called, will change the value of
/// [debugProfileBuildsEnabled], which adds [Timeline] events for every widget
/// built.
///
/// See also:
///
/// * [debugProfileBuildsEnabled], which is the flag that this service extension
/// exposes.
/// * [WidgetsBinding.initServiceExtensions], where the service extension is
/// registered.
profileWidgetBuilds,
/// Name of service extension that, when called, will change the value of
/// [debugProfileBuildsEnabledUserWidgets], which adds [Timeline] events for
/// every user-created widget built.
///
/// See also:
/// * [debugProfileBuildsEnabledUserWidgets], which is the flag that this
/// service extension exposes.
/// * [WidgetsBinding.initServiceExtensions], where the service extension is
/// registered.
profileUserWidgetBuilds,
/// Name of service extension that, when called, will change the value of
/// [WidgetsApp.debugAllowBannerOverride], which controls the visibility of the
/// debug banner for debug mode apps.
///
/// See also:
///
/// * [WidgetsApp.debugAllowBannerOverride], which is the flag that this service
/// extension exposes.
/// * [WidgetsBinding.initServiceExtensions], where the service extension is
/// registered.
debugAllowBanner,
}
/// Service extension constants for the Widget Inspector.
///
/// These constants will be used when registering service extensions in the
/// framework, and they will also be used by tools and services that call these
/// service extensions.
///
/// The String value for each of these extension names should be accessed by
/// calling the `.name` property on the enum value.
enum WidgetInspectorServiceExtensions {
/// Name of service extension that, when called, will determine whether
/// [FlutterError] messages will be presented using a structured format.
///
/// See also:
///
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
structuredErrors,
/// Name of service extension that, when called, will change the value of
/// [WidgetsBinding.debugShowWidgetInspectorOverride], which controls whether the
/// on-device widget inspector is visible.
///
/// See also:
/// * [WidgetsBinding.debugShowWidgetInspectorOverride], which is the flag that
/// this service extension exposes.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
show,
/// Name of service extension that, when called, determines
/// whether a callback is invoked for every dirty [Widget] built each frame.
///
/// See also:
///
/// * [debugOnRebuildDirtyWidget], which is the nullable callback that is
/// called for every dirty widget built per frame
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
trackRebuildDirtyWidgets,
/// Name of service extension that, when called, determines whether
/// a callback is invoked for every [RenderObject] painted each frame.
///
/// See also:
///
/// * [debugOnProfilePaint], which is the nullable callback that is called for
/// every dirty widget built per frame
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
trackRepaintWidgets,
/// Name of service extension that, when called, will clear all
/// [WidgetInspectorService] object references in all groups.
///
/// See also:
///
/// * [WidgetInspectorService.disposeAllGroups], the method that this service
/// extension calls.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
disposeAllGroups,
/// Name of service extension that, when called, will clear all
/// [WidgetInspectorService] object references in a group.
///
/// See also:
///
/// * [WidgetInspectorService.disposeGroup], the method that this service
/// extension calls.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
disposeGroup,
/// Name of service extension that, when called, returns whether it is
/// appropriate to display the Widget tree in the inspector, which is only
/// true after the application has rendered its first frame.
///
/// See also:
///
/// * [WidgetInspectorService.isWidgetTreeReady], the method that this service
/// extension calls.
/// * [WidgetsBinding.debugDidSendFirstFrameEvent], which stores the
/// value of whether the first frame has been rendered.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
isWidgetTreeReady,
/// Name of service extension that, when called, will remove the object with
/// the specified `id` from the specified object group.
///
/// See also:
///
/// * [WidgetInspectorService.disposeId], the method that this service
/// extension calls.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
disposeId,
/// Name of service extension that, when called, will set the list of
/// directories that should be considered part of the local project for the
/// Widget inspector summary tree.
///
/// See also:
///
/// * [WidgetInspectorService.addPubRootDirectories], which should be used in
/// place of this method to add directories.
/// * [WidgetInspectorService.removePubRootDirectories], which should be used
/// in place of this method to remove directories.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
@Deprecated(
'Use addPubRootDirectories instead. '
'This feature was deprecated after v3.18.0-2.0.pre.',
)
setPubRootDirectories,
/// Name of service extension that, when called, will add a list of
/// directories that should be considered part of the local project for the
/// Widget inspector summary tree.
///
/// See also:
///
/// * [WidgetInspectorService.addPubRootDirectories], the method that this
/// service extension calls.
/// * [WidgetInspectorService.removePubRootDirectories], which should be used
/// to remove directories.
/// * [WidgetInspectorService.pubRootDirectories], which should be used
/// to return the active list of directories.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
addPubRootDirectories,
/// Name of service extension that, when called, will remove a list of
/// directories that should no longer be considered part of the local project
/// for the Widget inspector summary tree.
///
/// See also:
///
/// * [WidgetInspectorService.removePubRootDirectories], the method that this
/// service extension calls.
/// * [WidgetInspectorService.addPubRootDirectories], which should be used
/// to add directories.
/// * [WidgetInspectorService.pubRootDirectories], which should be used
/// to return the active list of directories.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
removePubRootDirectories,
/// Name of service extension that, when called, will return the list of
/// directories that are considered part of the local project
/// for the Widget inspector summary tree.
///
/// See also:
///
/// * [WidgetInspectorService.pubRootDirectories], the method that this
/// service extension calls.
/// * [WidgetInspectorService.addPubRootDirectories], which should be used
/// to add directories.
/// * [WidgetInspectorService.removePubRootDirectories], which should be used
/// to remove directories.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
getPubRootDirectories,
/// Name of service extension that, when called, will set the
/// [WidgetInspector] selection to the object matching the specified id and
/// will return whether the selection was changed.
///
/// See also:
///
/// * [WidgetInspectorService.setSelectionById], the method that this service
/// extension calls.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
setSelectionById,
/// Name of service extension that, when called, will retrieve the chain of
/// [DiagnosticsNode] instances form the root of the tree to the [Element] or
/// [RenderObject] matching the specified id, passed as an argument.
///
/// See also:
///
/// * [WidgetInspectorService.getParentChain], which returns a json encoded
/// String representation of this data.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
getParentChain,
/// Name of service extension that, when called, will return the properties
/// for the [DiagnosticsNode] object matching the specified id, passed as an
/// argument.
///
/// See also:
///
/// * [WidgetInspectorService.getProperties], which returns a json encoded
/// String representation of this data.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
getProperties,
/// Name of service extension that, when called, will return the children
/// for the [DiagnosticsNode] object matching the specified id, passed as an
/// argument.
///
/// See also:
///
/// * [WidgetInspectorService.getChildren], which returns a json encoded
/// String representation of this data.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
getChildren,
/// Name of service extension that, when called, will return the children
/// created by user code for the [DiagnosticsNode] object matching the
/// specified id, passed as an argument.
///
/// See also:
///
/// * [WidgetInspectorService.getChildrenSummaryTree], which returns a json
/// encoded String representation of this data.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
getChildrenSummaryTree,
/// Name of service extension that, when called, will return all children and
/// their properties for the [DiagnosticsNode] object matching the specified
/// id, passed as an argument.
///
/// See also:
///
/// * [WidgetInspectorService.getChildrenDetailsSubtree], which returns a json
/// encoded String representation of this data.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
getChildrenDetailsSubtree,
/// Name of service extension that, when called, will return the
/// [DiagnosticsNode] data for the root [Element].
///
/// See also:
///
/// * [WidgetInspectorService.getRootWidget], which returns a json encoded
/// String representation of this data.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
getRootWidget,
/// Name of service extension that, when called, will return the
/// [DiagnosticsNode] data for the root [Element] of the summary tree, which
/// only includes [Element]s that were created by user code.
///
/// See also:
///
/// * [WidgetInspectorService.getRootWidgetSummaryTree], which returns a json
/// encoded String representation of this data.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
getRootWidgetSummaryTree,
/// Name of service extension that, when called, will return the
/// [DiagnosticsNode] data for the root [Element] of the summary tree with
/// text previews included.
///
/// The summary tree only includes [Element]s that were created by user code.
/// Text previews will only be available for [Element]s with a corresponding
/// [RenderObject] of type [RenderParagraph].
///
/// See also:
///
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
getRootWidgetSummaryTreeWithPreviews,
/// Name of service extension that, when called, will return the details
/// subtree, which includes properties, rooted at the [DiagnosticsNode] object
/// matching the specified id and the having a size matching the specified
/// subtree depth, both passed as arguments.
///
/// See also:
///
/// * [WidgetInspectorService.getDetailsSubtree], the method that this service
/// extension calls.
/// * [WidgetInspectorService.getDetailsSubtree], which returns a json
/// encoded String representation of this data.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
getDetailsSubtree,
/// Name of service extension that, when called, will return the
/// [DiagnosticsNode] data for the currently selected [Element].
///
/// See also:
///
/// * [WidgetInspectorService.getSelectedWidget], which returns a json
/// encoded String representation of this data.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
getSelectedWidget,
/// Name of service extension that, when called, will return the
/// [DiagnosticsNode] data for the currently selected [Element] in the summary
/// tree, which only includes [Element]s created in user code.
///
/// If the selected [Element] does not exist in the summary tree, the first
/// ancestor in the summary tree for the currently selected [Element] will be
/// returned.
///
/// See also:
///
/// * [WidgetInspectorService.getSelectedSummaryWidget], which returns a json
/// encoded String representation of this data.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
getSelectedSummaryWidget,
/// Name of service extension that, when called, will return whether [Widget]
/// creation locations are available.
///
/// See also:
///
/// * [WidgetInspectorService.isWidgetCreationTracked], the method that this
/// service extension calls.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
isWidgetCreationTracked,
/// Name of service extension that, when called, will return a base64 encoded
/// image of the [RenderObject] or [Element] matching the specified 'id`,
/// passed as an argument, and sized at the specified 'width' and 'height'
/// values, also passed as arguments.
///
/// See also:
///
/// * [WidgetInspectorService.screenshot], the method that this service
/// extension calls.
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
screenshot,
/// Name of service extension that, when called, will return the
/// [DiagnosticsNode] data for the currently selected [Element] and will
/// include information about the [Element]'s layout properties.
///
/// See also:
///
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
getLayoutExplorerNode,
/// Name of service extension that, when called, will set the [FlexFit] value
/// for the [FlexParentData] of the [RenderObject] matching the specified
/// `id`, passed as an argument.
///
/// See also:
///
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
setFlexFit,
/// Name of service extension that, when called, will set the flex value
/// for the [FlexParentData] of the [RenderObject] matching the specified
/// `id`, passed as an argument.
///
/// See also:
///
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
setFlexFactor,
/// Name of service extension that, when called, will set the
/// [MainAxisAlignment] and [CrossAxisAlignment] values for the [RenderFlex]
/// matching the specified `id`, passed as an argument.
///
/// The [MainAxisAlignment] and [CrossAxisAlignment] values will be passed as
/// arguments `mainAxisAlignment` and `crossAxisAlignment`, respectively.
///
/// See also:
///
/// * [WidgetInspectorService.initServiceExtensions], where the service
/// extension is registered.
setFlexProperties,
}
| flutter/packages/flutter/lib/src/widgets/service_extensions.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/service_extensions.dart",
"repo_id": "flutter",
"token_count": 5017
} | 675 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:collection';
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'basic.dart';
import 'debug.dart';
import 'framework.dart';
import 'image.dart';
export 'package:flutter/rendering.dart' show
FixedColumnWidth,
FlexColumnWidth,
FractionColumnWidth,
IntrinsicColumnWidth,
MaxColumnWidth,
MinColumnWidth,
TableBorder,
TableCellVerticalAlignment,
TableColumnWidth;
/// A horizontal group of cells in a [Table].
///
/// Every row in a table must have the same number of children.
///
/// The alignment of individual cells in a row can be controlled using a
/// [TableCell].
@immutable
class TableRow {
/// Creates a row in a [Table].
const TableRow({ this.key, this.decoration, this.children = const <Widget>[]});
/// An identifier for the row.
final LocalKey? key;
/// A decoration to paint behind this row.
///
/// Row decorations fill the horizontal and vertical extent of each row in
/// the table, unlike decorations for individual cells, which might not fill
/// either.
final Decoration? decoration;
/// The widgets that comprise the cells in this row.
///
/// Children may be wrapped in [TableCell] widgets to provide per-cell
/// configuration to the [Table], but children are not required to be wrapped
/// in [TableCell] widgets.
final List<Widget> children;
@override
String toString() {
final StringBuffer result = StringBuffer();
result.write('TableRow(');
if (key != null) {
result.write('$key, ');
}
if (decoration != null) {
result.write('$decoration, ');
}
if (children.isEmpty) {
result.write('no children');
} else {
result.write('$children');
}
result.write(')');
return result.toString();
}
}
class _TableElementRow {
const _TableElementRow({ this.key, required this.children });
final LocalKey? key;
final List<Element> children;
}
/// A widget that uses the table layout algorithm for its children.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=_lbE0wsVZSw}
///
/// {@tool dartpad}
/// This sample shows a [Table] with borders, multiple types of column widths
/// and different vertical cell alignments.
///
/// ** See code in examples/api/lib/widgets/table/table.0.dart **
/// {@end-tool}
///
/// If you only have one row, the [Row] widget is more appropriate. If you only
/// have one column, the [SliverList] or [Column] widgets will be more
/// appropriate.
///
/// Rows size vertically based on their contents. To control the individual
/// column widths, use the [columnWidths] property to specify a
/// [TableColumnWidth] for each column. If [columnWidths] is null, or there is a
/// null entry for a given column in [columnWidths], the table uses the
/// [defaultColumnWidth] instead.
///
/// By default, [defaultColumnWidth] is a [FlexColumnWidth]. This
/// [TableColumnWidth] divides up the remaining space in the horizontal axis to
/// determine the column width. If wrapping a [Table] in a horizontal
/// [ScrollView], choose a different [TableColumnWidth], such as
/// [FixedColumnWidth].
///
/// For more details about the table layout algorithm, see [RenderTable].
/// To control the alignment of children, see [TableCell].
///
/// See also:
///
/// * The [catalog of layout widgets](https://flutter.dev/widgets/layout/).
class Table extends RenderObjectWidget {
/// Creates a table.
Table({
super.key,
this.children = const <TableRow>[],
this.columnWidths,
this.defaultColumnWidth = const FlexColumnWidth(),
this.textDirection,
this.border,
this.defaultVerticalAlignment = TableCellVerticalAlignment.top,
this.textBaseline, // NO DEFAULT: we don't know what the text's baseline should be
}) : assert(defaultVerticalAlignment != TableCellVerticalAlignment.baseline || textBaseline != null, 'textBaseline is required if you specify the defaultVerticalAlignment with TableCellVerticalAlignment.baseline'),
assert(() {
if (children.any((TableRow row1) => row1.key != null && children.any((TableRow row2) => row1 != row2 && row1.key == row2.key))) {
throw FlutterError(
'Two or more TableRow children of this Table had the same key.\n'
'All the keyed TableRow children of a Table must have different Keys.',
);
}
return true;
}()),
assert(() {
if (children.isNotEmpty) {
final int cellCount = children.first.children.length;
if (children.any((TableRow row) => row.children.length != cellCount)) {
throw FlutterError(
'Table contains irregular row lengths.\n'
'Every TableRow in a Table must have the same number of children, so that every cell is filled. '
'Otherwise, the table will contain holes.',
);
}
if (children.any((TableRow row) => row.children.isEmpty)) {
throw FlutterError(
'One or more TableRow have no children.\n'
'Every TableRow in a Table must have at least one child, so there is no empty row. ',
);
}
}
return true;
}()),
_rowDecorations = children.any((TableRow row) => row.decoration != null)
? children.map<Decoration?>((TableRow row) => row.decoration).toList(growable: false)
: null {
assert(() {
final List<Widget> flatChildren = children.expand<Widget>((TableRow row) => row.children).toList(growable: false);
return !debugChildrenHaveDuplicateKeys(this, flatChildren, message:
'Two or more cells in this Table contain widgets with the same key.\n'
'Every widget child of every TableRow in a Table must have different keys. The cells of a Table are '
'flattened out for processing, so separate cells cannot have duplicate keys even if they are in '
'different rows.',
);
}());
}
/// The rows of the table.
///
/// Every row in a table must have the same number of children.
final List<TableRow> children;
/// How the horizontal extents of the columns of this table should be determined.
///
/// If the [Map] has a null entry for a given column, the table uses the
/// [defaultColumnWidth] instead. By default, that uses flex sizing to
/// distribute free space equally among the columns.
///
/// The [FixedColumnWidth] class can be used to specify a specific width in
/// pixels. That is the cheapest way to size a table's columns.
///
/// The layout performance of the table depends critically on which column
/// sizing algorithms are used here. In particular, [IntrinsicColumnWidth] is
/// quite expensive because it needs to measure each cell in the column to
/// determine the intrinsic size of the column.
///
/// The keys of this map (column indexes) are zero-based.
///
/// If this is set to null, then an empty map is assumed.
final Map<int, TableColumnWidth>? columnWidths;
/// How to determine with widths of columns that don't have an explicit sizing
/// algorithm.
///
/// Specifically, the [defaultColumnWidth] is used for column `i` if
/// `columnWidths[i]` is null. Defaults to [FlexColumnWidth], which will
/// divide the remaining horizontal space up evenly between columns of the
/// same type [TableColumnWidth].
///
/// A [Table] in a horizontal [ScrollView] must use a [FixedColumnWidth], or
/// an [IntrinsicColumnWidth] as the horizontal space is infinite.
final TableColumnWidth defaultColumnWidth;
/// The direction in which the columns are ordered.
///
/// Defaults to the ambient [Directionality].
final TextDirection? textDirection;
/// The style to use when painting the boundary and interior divisions of the table.
final TableBorder? border;
/// How cells that do not explicitly specify a vertical alignment are aligned vertically.
///
/// Cells may specify a vertical alignment by wrapping their contents in a
/// [TableCell] widget.
final TableCellVerticalAlignment defaultVerticalAlignment;
/// The text baseline to use when aligning rows using [TableCellVerticalAlignment.baseline].
///
/// This must be set if using baseline alignment. There is no default because there is no
/// way for the framework to know the correct baseline _a priori_.
final TextBaseline? textBaseline;
final List<Decoration?>? _rowDecorations;
@override
RenderObjectElement createElement() => _TableElement(this);
@override
RenderTable createRenderObject(BuildContext context) {
assert(debugCheckHasDirectionality(context));
return RenderTable(
columns: children.isNotEmpty ? children[0].children.length : 0,
rows: children.length,
columnWidths: columnWidths,
defaultColumnWidth: defaultColumnWidth,
textDirection: textDirection ?? Directionality.of(context),
border: border,
rowDecorations: _rowDecorations,
configuration: createLocalImageConfiguration(context),
defaultVerticalAlignment: defaultVerticalAlignment,
textBaseline: textBaseline,
);
}
@override
void updateRenderObject(BuildContext context, RenderTable renderObject) {
assert(debugCheckHasDirectionality(context));
assert(renderObject.columns == (children.isNotEmpty ? children[0].children.length : 0));
assert(renderObject.rows == children.length);
renderObject
..columnWidths = columnWidths
..defaultColumnWidth = defaultColumnWidth
..textDirection = textDirection ?? Directionality.of(context)
..border = border
..rowDecorations = _rowDecorations
..configuration = createLocalImageConfiguration(context)
..defaultVerticalAlignment = defaultVerticalAlignment
..textBaseline = textBaseline;
}
}
class _TableElement extends RenderObjectElement {
_TableElement(Table super.widget);
@override
RenderTable get renderObject => super.renderObject as RenderTable;
List<_TableElementRow> _children = const<_TableElementRow>[];
bool _doingMountOrUpdate = false;
@override
void mount(Element? parent, Object? newSlot) {
assert(!_doingMountOrUpdate);
_doingMountOrUpdate = true;
super.mount(parent, newSlot);
int rowIndex = -1;
_children = (widget as Table).children.map<_TableElementRow>((TableRow row) {
int columnIndex = 0;
rowIndex += 1;
return _TableElementRow(
key: row.key,
children: row.children.map<Element>((Widget child) {
return inflateWidget(child, _TableSlot(columnIndex++, rowIndex));
}).toList(growable: false),
);
}).toList(growable: false);
_updateRenderObjectChildren();
assert(_doingMountOrUpdate);
_doingMountOrUpdate = false;
}
@override
void insertRenderObjectChild(RenderBox child, _TableSlot slot) {
renderObject.setupParentData(child);
// Once [mount]/[update] are done, the children are getting set all at once
// in [_updateRenderObjectChildren].
if (!_doingMountOrUpdate) {
renderObject.setChild(slot.column, slot.row, child);
}
}
@override
void moveRenderObjectChild(RenderBox child, _TableSlot oldSlot, _TableSlot newSlot) {
assert(_doingMountOrUpdate);
// Child gets moved at the end of [update] in [_updateRenderObjectChildren].
}
@override
void removeRenderObjectChild(RenderBox child, _TableSlot slot) {
renderObject.setChild(slot.column, slot.row, null);
}
final Set<Element> _forgottenChildren = HashSet<Element>();
@override
void update(Table newWidget) {
assert(!_doingMountOrUpdate);
_doingMountOrUpdate = true;
final Map<LocalKey, List<Element>> oldKeyedRows = <LocalKey, List<Element>>{};
for (final _TableElementRow row in _children) {
if (row.key != null) {
oldKeyedRows[row.key!] = row.children;
}
}
final Iterator<_TableElementRow> oldUnkeyedRows = _children.where((_TableElementRow row) => row.key == null).iterator;
final List<_TableElementRow> newChildren = <_TableElementRow>[];
final Set<List<Element>> taken = <List<Element>>{};
for (int rowIndex = 0; rowIndex < newWidget.children.length; rowIndex++) {
final TableRow row = newWidget.children[rowIndex];
List<Element> oldChildren;
if (row.key != null && oldKeyedRows.containsKey(row.key)) {
oldChildren = oldKeyedRows[row.key]!;
taken.add(oldChildren);
} else if (row.key == null && oldUnkeyedRows.moveNext()) {
oldChildren = oldUnkeyedRows.current.children;
} else {
oldChildren = const <Element>[];
}
final List<_TableSlot> slots = List<_TableSlot>.generate(
row.children.length,
(int columnIndex) => _TableSlot(columnIndex, rowIndex),
);
newChildren.add(_TableElementRow(
key: row.key,
children: updateChildren(oldChildren, row.children, forgottenChildren: _forgottenChildren, slots: slots),
));
}
while (oldUnkeyedRows.moveNext()) {
updateChildren(oldUnkeyedRows.current.children, const <Widget>[], forgottenChildren: _forgottenChildren);
}
for (final List<Element> oldChildren in oldKeyedRows.values.where((List<Element> list) => !taken.contains(list))) {
updateChildren(oldChildren, const <Widget>[], forgottenChildren: _forgottenChildren);
}
_children = newChildren;
_updateRenderObjectChildren();
_forgottenChildren.clear();
super.update(newWidget);
assert(widget == newWidget);
assert(_doingMountOrUpdate);
_doingMountOrUpdate = false;
}
void _updateRenderObjectChildren() {
renderObject.setFlatChildren(
_children.isNotEmpty ? _children[0].children.length : 0,
_children.expand<RenderBox>((_TableElementRow row) {
return row.children.map<RenderBox>((Element child) {
final RenderBox box = child.renderObject! as RenderBox;
return box;
});
}).toList(),
);
}
@override
void visitChildren(ElementVisitor visitor) {
for (final Element child in _children.expand<Element>((_TableElementRow row) => row.children)) {
if (!_forgottenChildren.contains(child)) {
visitor(child);
}
}
}
@override
bool forgetChild(Element child) {
_forgottenChildren.add(child);
super.forgetChild(child);
return true;
}
}
/// A widget that controls how a child of a [Table] is aligned.
///
/// A [TableCell] widget must be a descendant of a [Table], and the path from
/// the [TableCell] widget to its enclosing [Table] must contain only
/// [TableRow]s, [StatelessWidget]s, or [StatefulWidget]s (not
/// other kinds of widgets, like [RenderObjectWidget]s).
///
/// To create an empty [TableCell], provide a [SizedBox.shrink]
/// as the [child].
class TableCell extends ParentDataWidget<TableCellParentData> {
/// Creates a widget that controls how a child of a [Table] is aligned.
const TableCell({
super.key,
this.verticalAlignment,
required super.child,
});
/// How this cell is aligned vertically.
final TableCellVerticalAlignment? verticalAlignment;
@override
void applyParentData(RenderObject renderObject) {
final TableCellParentData parentData = renderObject.parentData! as TableCellParentData;
if (parentData.verticalAlignment != verticalAlignment) {
parentData.verticalAlignment = verticalAlignment;
final RenderObject? targetParent = renderObject.parent;
if (targetParent is RenderObject) {
targetParent.markNeedsLayout();
}
}
}
@override
Type get debugTypicalAncestorWidgetClass => Table;
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(EnumProperty<TableCellVerticalAlignment>('verticalAlignment', verticalAlignment));
}
}
@immutable
class _TableSlot with Diagnosticable {
const _TableSlot(this.column, this.row);
final int column;
final int row;
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is _TableSlot
&& column == other.column
&& row == other.row;
}
@override
int get hashCode => Object.hash(column, row);
@override
void debugFillProperties(DiagnosticPropertiesBuilder properties) {
super.debugFillProperties(properties);
properties.add(IntProperty('x', column));
properties.add(IntProperty('y', row));
}
}
| flutter/packages/flutter/lib/src/widgets/table.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/table.dart",
"repo_id": "flutter",
"token_count": 5527
} | 676 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'framework.dart';
/// Builds a [Widget] when given a concrete value of a [ValueListenable<T>].
///
/// If the `child` parameter provided to the [ValueListenableBuilder] is not
/// null, the same `child` widget is passed back to this [ValueWidgetBuilder]
/// and should typically be incorporated in the returned widget tree.
///
/// See also:
///
/// * [ValueListenableBuilder], a widget which invokes this builder each time
/// a [ValueListenable] changes value.
typedef ValueWidgetBuilder<T> = Widget Function(BuildContext context, T value, Widget? child);
/// A widget whose content stays synced with a [ValueListenable].
///
/// Given a [ValueListenable<T>] and a [builder] which builds widgets from
/// concrete values of `T`, this class will automatically register itself as a
/// listener of the [ValueListenable] and call the [builder] with updated values
/// when the value changes.
///
/// {@youtube 560 315 https://www.youtube.com/watch?v=s-ZG-jS5QHQ}
///
/// ## Performance optimizations
///
/// If your [builder] function contains a subtree that does not depend on the
/// value of the [ValueListenable], it's more efficient to build that subtree
/// once instead of rebuilding it on every animation tick.
///
/// If you pass the pre-built subtree as the [child] parameter, the
/// [ValueListenableBuilder] will pass it back to your [builder] function so
/// that you can incorporate it into your build.
///
/// Using this pre-built child is entirely optional, but can improve
/// performance significantly in some cases and is therefore a good practice.
///
/// {@tool snippet}
///
/// This sample shows how you could use a [ValueListenableBuilder] instead of
/// setting state on the whole [Scaffold] in the default `flutter create` app.
///
/// ```dart
/// class MyHomePage extends StatefulWidget {
/// const MyHomePage({super.key, required this.title});
/// final String title;
///
/// @override
/// State<MyHomePage> createState() => _MyHomePageState();
/// }
///
/// class _MyHomePageState extends State<MyHomePage> {
/// final ValueNotifier<int> _counter = ValueNotifier<int>(0);
/// final Widget goodJob = const Text('Good job!');
/// @override
/// Widget build(BuildContext context) {
/// return Scaffold(
/// appBar: AppBar(
/// title: Text(widget.title)
/// ),
/// body: Center(
/// child: Column(
/// mainAxisAlignment: MainAxisAlignment.center,
/// children: <Widget>[
/// const Text('You have pushed the button this many times:'),
/// ValueListenableBuilder<int>(
/// builder: (BuildContext context, int value, Widget? child) {
/// // This builder will only get called when the _counter
/// // is updated.
/// return Row(
/// mainAxisAlignment: MainAxisAlignment.spaceEvenly,
/// children: <Widget>[
/// Text('$value'),
/// child!,
/// ],
/// );
/// },
/// valueListenable: _counter,
/// // The child parameter is most helpful if the child is
/// // expensive to build and does not depend on the value from
/// // the notifier.
/// child: goodJob,
/// )
/// ],
/// ),
/// ),
/// floatingActionButton: FloatingActionButton(
/// child: const Icon(Icons.plus_one),
/// onPressed: () => _counter.value += 1,
/// ),
/// );
/// }
/// }
/// ```
/// {@end-tool}
///
/// See also:
///
/// * [AnimatedBuilder], which also triggers rebuilds from a [Listenable]
/// without passing back a specific value from a [ValueListenable].
/// * [NotificationListener], which lets you rebuild based on [Notification]
/// coming from its descendant widgets rather than a [ValueListenable] that
/// you have a direct reference to.
/// * [StreamBuilder], where a builder can depend on a [Stream] rather than
/// a [ValueListenable] for more advanced use cases.
class ValueListenableBuilder<T> extends StatefulWidget {
/// Creates a [ValueListenableBuilder].
///
/// The [child] is optional but is good practice to use if part of the widget
/// subtree does not depend on the value of the [valueListenable].
const ValueListenableBuilder({
super.key,
required this.valueListenable,
required this.builder,
this.child,
});
/// The [ValueListenable] whose value you depend on in order to build.
///
/// This widget does not ensure that the [ValueListenable]'s value is not
/// null, therefore your [builder] may need to handle null values.
final ValueListenable<T> valueListenable;
/// A [ValueWidgetBuilder] which builds a widget depending on the
/// [valueListenable]'s value.
///
/// Can incorporate a [valueListenable] value-independent widget subtree
/// from the [child] parameter into the returned widget tree.
final ValueWidgetBuilder<T> builder;
/// A [valueListenable]-independent widget which is passed back to the [builder].
///
/// This argument is optional and can be null if the entire widget subtree the
/// [builder] builds depends on the value of the [valueListenable]. For
/// example, in the case where the [valueListenable] is a [String] and the
/// [builder] returns a [Text] widget with the current [String] value, there
/// would be no useful [child].
final Widget? child;
@override
State<StatefulWidget> createState() => _ValueListenableBuilderState<T>();
}
class _ValueListenableBuilderState<T> extends State<ValueListenableBuilder<T>> {
late T value;
@override
void initState() {
super.initState();
value = widget.valueListenable.value;
widget.valueListenable.addListener(_valueChanged);
}
@override
void didUpdateWidget(ValueListenableBuilder<T> oldWidget) {
super.didUpdateWidget(oldWidget);
if (oldWidget.valueListenable != widget.valueListenable) {
oldWidget.valueListenable.removeListener(_valueChanged);
value = widget.valueListenable.value;
widget.valueListenable.addListener(_valueChanged);
}
}
@override
void dispose() {
widget.valueListenable.removeListener(_valueChanged);
super.dispose();
}
void _valueChanged() {
setState(() { value = widget.valueListenable.value; });
}
@override
Widget build(BuildContext context) {
return widget.builder(context, value, widget.child);
}
}
| flutter/packages/flutter/lib/src/widgets/value_listenable_builder.dart/0 | {
"file_path": "flutter/packages/flutter/lib/src/widgets/value_listenable_builder.dart",
"repo_id": "flutter",
"token_count": 2226
} | 677 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('Animation created from ValueListenable', () {
final ValueNotifier<double> listenable = ValueNotifier<double>(0.0);
final Animation<double> animation = Animation<double>.fromValueListenable(listenable);
expect(animation.status, AnimationStatus.forward);
expect(animation.value, 0.0);
listenable.value = 1.0;
expect(animation.value, 1.0);
bool listenerCalled = false;
void listener() {
listenerCalled = true;
}
animation.addListener(listener);
listenable.value = 0.5;
expect(listenerCalled, true);
listenerCalled = false;
animation.removeListener(listener);
listenable.value = 0.2;
expect(listenerCalled, false);
});
test('Animation created from ValueListenable can transform value', () {
final ValueNotifier<double> listenable = ValueNotifier<double>(0.0);
final Animation<double> animation = Animation<double>.fromValueListenable(listenable, transformer: (double input) {
return input / 10;
});
expect(animation.status, AnimationStatus.forward);
expect(animation.value, 0.0);
listenable.value = 10.0;
expect(animation.value, 1.0);
});
test('Animation created from ValueListenable can be transformed via drive', () {
final ValueNotifier<double> listenable = ValueNotifier<double>(0.0);
final Animation<double> animation = Animation<double>.fromValueListenable(listenable);
final Animation<Offset> offset = animation.drive(Animatable<Offset>.fromCallback((double value) {
return Offset(0.0, value);
}));
expect(offset.value, Offset.zero);
expect(offset.status, AnimationStatus.forward);
listenable.value = 10;
expect(offset.value, const Offset(0.0, 10.0));
bool listenerCalled = false;
void listener() {
listenerCalled = true;
}
offset.addListener(listener);
listenable.value = 0.5;
expect(listenerCalled, true);
listenerCalled = false;
offset.removeListener(listener);
listenable.value = 0.2;
expect(listenerCalled, false);
});
}
| flutter/packages/flutter/test/animation/animation_from_listener_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/animation/animation_from_listener_test.dart",
"repo_id": "flutter",
"token_count": 776
} | 678 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/semantics_tester.dart';
const TextStyle testStyle = TextStyle(
fontSize: 10.0,
letterSpacing: 0.0,
);
void main() {
testWidgets('Default layout minimum size', (WidgetTester tester) async {
await tester.pumpWidget(
boilerplate(child: const CupertinoButton(
onPressed: null,
child: Text('X', style: testStyle),
)),
);
final RenderBox buttonBox = tester.renderObject(find.byType(CupertinoButton));
expect(
buttonBox.size,
// 1 10px character + 16px * 2 is smaller than the default 44px minimum.
const Size.square(44.0),
);
});
testWidgets('Minimum size parameter', (WidgetTester tester) async {
const double minSize = 60.0;
await tester.pumpWidget(
boilerplate(child: const CupertinoButton(
onPressed: null,
minSize: minSize,
child: Text('X', style: testStyle),
)),
);
final RenderBox buttonBox = tester.renderObject(find.byType(CupertinoButton));
expect(
buttonBox.size,
// 1 10px character + 16px * 2 is smaller than defined 60.0px minimum
const Size.square(minSize),
);
});
testWidgets('Size grows with text', (WidgetTester tester) async {
await tester.pumpWidget(
boilerplate(child: const CupertinoButton(
onPressed: null,
child: Text('XXXX', style: testStyle),
)),
);
final RenderBox buttonBox = tester.renderObject(find.byType(CupertinoButton));
expect(
buttonBox.size.width,
// 4 10px character + 16px * 2 = 72.
72.0,
);
});
// TODO(LongCatIsLoong): Uncomment once https://github.com/flutter/flutter/issues/44115
// is fixed.
/*
testWidgets(
'CupertinoButton.filled default color contrast meets guideline',
(WidgetTester tester) async {
// The native color combination systemBlue text over white background fails
// to pass the color contrast guideline.
//await tester.pumpWidget(
// CupertinoTheme(
// data: const CupertinoThemeData(),
// child: Directionality(
// textDirection: TextDirection.ltr,
// child: CupertinoButton.filled(
// child: const Text('Button'),
// onPressed: () {},
// ),
// ),
// ),
//);
//await expectLater(tester, meetsGuideline(textContrastGuideline));
await tester.pumpWidget(
CupertinoApp(
theme: const CupertinoThemeData(brightness: Brightness.dark),
home: CupertinoPageScaffold(
child: CupertinoButton.filled(
child: const Text('Button'),
onPressed: () {},
),
),
),
);
await expectLater(tester, meetsGuideline(textContrastGuideline));
});
*/
testWidgets('Button child alignment', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: CupertinoButton(
onPressed: () { },
child: const Text('button'),
),
),
);
Align align = tester.firstWidget<Align>(find.ancestor(of: find.text('button'), matching: find.byType(Align)));
expect(align.alignment, Alignment.center); // default
await tester.pumpWidget(
CupertinoApp(
home: CupertinoButton(
alignment: Alignment.centerLeft,
onPressed: () { },
child: const Text('button'),
),
),
);
align = tester.firstWidget<Align>(find.ancestor(of: find.text('button'), matching: find.byType(Align)));
expect(align.alignment, Alignment.centerLeft);
});
testWidgets('Button with background is wider', (WidgetTester tester) async {
await tester.pumpWidget(boilerplate(child: const CupertinoButton(
onPressed: null,
color: Color(0xFFFFFFFF),
child: Text('X', style: testStyle),
)));
final RenderBox buttonBox = tester.renderObject(find.byType(CupertinoButton));
expect(
buttonBox.size.width,
// 1 10px character + 64 * 2 = 138 for buttons with background.
138.0,
);
});
testWidgets('Custom padding', (WidgetTester tester) async {
await tester.pumpWidget(boilerplate(child: const CupertinoButton(
onPressed: null,
padding: EdgeInsets.all(100.0),
child: Text('X', style: testStyle),
)));
final RenderBox buttonBox = tester.renderObject(find.byType(CupertinoButton));
expect(
buttonBox.size,
const Size.square(210.0),
);
});
testWidgets('Button takes taps', (WidgetTester tester) async {
bool value = false;
await tester.pumpWidget(
StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return boilerplate(
child: CupertinoButton(
child: const Text('Tap me'),
onPressed: () {
setState(() {
value = true;
});
},
),
);
},
),
);
expect(value, isFalse);
// No animating by default.
expect(SchedulerBinding.instance.transientCallbackCount, equals(0));
await tester.tap(find.byType(CupertinoButton));
expect(value, isTrue);
// Animates.
expect(SchedulerBinding.instance.transientCallbackCount, equals(1));
});
testWidgets("Disabled button doesn't animate", (WidgetTester tester) async {
await tester.pumpWidget(boilerplate(child: const CupertinoButton(
onPressed: null,
child: Text('Tap me'),
)));
expect(SchedulerBinding.instance.transientCallbackCount, equals(0));
await tester.tap(find.byType(CupertinoButton));
// Still doesn't animate.
expect(SchedulerBinding.instance.transientCallbackCount, equals(0));
});
testWidgets('Enabled button animates', (WidgetTester tester) async {
await tester.pumpWidget(boilerplate(child: CupertinoButton(
child: const Text('Tap me'),
onPressed: () { },
)));
await tester.tap(find.byType(CupertinoButton));
// Enter animation.
await tester.pump();
FadeTransition transition = tester.firstWidget(find.byType(FadeTransition));
await tester.pump(const Duration(milliseconds: 50));
transition = tester.firstWidget(find.byType(FadeTransition));
expect(transition.opacity.value, moreOrLessEquals(0.403, epsilon: 0.001));
await tester.pump(const Duration(milliseconds: 100));
transition = tester.firstWidget(find.byType(FadeTransition));
expect(transition.opacity.value, moreOrLessEquals(0.400, epsilon: 0.001));
await tester.pump(const Duration(milliseconds: 50));
transition = tester.firstWidget(find.byType(FadeTransition));
expect(transition.opacity.value, moreOrLessEquals(0.650, epsilon: 0.001));
await tester.pump(const Duration(milliseconds: 50));
transition = tester.firstWidget(find.byType(FadeTransition));
expect(transition.opacity.value, moreOrLessEquals(0.894, epsilon: 0.001));
await tester.pump(const Duration(milliseconds: 50));
transition = tester.firstWidget(find.byType(FadeTransition));
expect(transition.opacity.value, moreOrLessEquals(0.988, epsilon: 0.001));
await tester.pump(const Duration(milliseconds: 50));
transition = tester.firstWidget(find.byType(FadeTransition));
expect(transition.opacity.value, moreOrLessEquals(1.0, epsilon: 0.001));
});
testWidgets('pressedOpacity defaults to 0.1', (WidgetTester tester) async {
await tester.pumpWidget(boilerplate(child: CupertinoButton(
child: const Text('Tap me'),
onPressed: () { },
)));
// Keep a "down" gesture on the button
final Offset center = tester.getCenter(find.byType(CupertinoButton));
final TestGesture gesture = await tester.startGesture(center);
await tester.pumpAndSettle();
// Check opacity
final FadeTransition opacity = tester.widget(find.descendant(
of: find.byType(CupertinoButton),
matching: find.byType(FadeTransition),
));
expect(opacity.opacity.value, 0.4);
// Finish gesture to release resources.
await gesture.up();
await tester.pumpAndSettle();
});
testWidgets('pressedOpacity parameter', (WidgetTester tester) async {
const double pressedOpacity = 0.5;
await tester.pumpWidget(boilerplate(child: CupertinoButton(
pressedOpacity: pressedOpacity,
child: const Text('Tap me'),
onPressed: () { },
)));
// Keep a "down" gesture on the button
final Offset center = tester.getCenter(find.byType(CupertinoButton));
final TestGesture gesture = await tester.startGesture(center);
await tester.pumpAndSettle();
// Check opacity
final FadeTransition opacity = tester.widget(find.descendant(
of: find.byType(CupertinoButton),
matching: find.byType(FadeTransition),
));
expect(opacity.opacity.value, pressedOpacity);
// Finish gesture to release resources.
await gesture.up();
await tester.pumpAndSettle();
});
testWidgets('Cupertino button is semantically a button', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(
boilerplate(
child: Center(
child: CupertinoButton(
onPressed: () { },
child: const Text('ABC'),
),
),
),
);
expect(semantics, hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics.rootChild(
actions: SemanticsAction.tap.index,
label: 'ABC',
flags: SemanticsFlag.isButton.index,
),
],
),
ignoreId: true,
ignoreRect: true,
ignoreTransform: true,
));
semantics.dispose();
});
testWidgets('Can specify colors', (WidgetTester tester) async {
await tester.pumpWidget(boilerplate(child: CupertinoButton(
color: const Color(0x000000FF),
disabledColor: const Color(0x0000FF00),
onPressed: () { },
child: const Text('Skeuomorph me'),
)));
BoxDecoration boxDecoration = tester.widget<DecoratedBox>(
find.widgetWithText(DecoratedBox, 'Skeuomorph me'),
).decoration as BoxDecoration;
expect(boxDecoration.color, const Color(0x000000FF));
await tester.pumpWidget(boilerplate(child: const CupertinoButton(
color: Color(0x000000FF),
disabledColor: Color(0x0000FF00),
onPressed: null,
child: Text('Skeuomorph me'),
)));
boxDecoration = tester.widget<DecoratedBox>(
find.widgetWithText(DecoratedBox, 'Skeuomorph me'),
).decoration as BoxDecoration;
expect(boxDecoration.color, const Color(0x0000FF00));
});
testWidgets('Can specify dynamic colors', (WidgetTester tester) async {
const Color bgColor = CupertinoDynamicColor.withBrightness(
color: Color(0xFF123456),
darkColor: Color(0xFF654321),
);
const Color inactive = CupertinoDynamicColor.withBrightness(
color: Color(0xFF111111),
darkColor: Color(0xFF222222),
);
await tester.pumpWidget(
MediaQuery(
data: const MediaQueryData(platformBrightness: Brightness.dark),
child: boilerplate(child: CupertinoButton(
color: bgColor,
disabledColor: inactive,
onPressed: () { },
child: const Text('Skeuomorph me'),
)),
),
);
BoxDecoration boxDecoration = tester.widget<DecoratedBox>(
find.widgetWithText(DecoratedBox, 'Skeuomorph me'),
).decoration as BoxDecoration;
expect(boxDecoration.color!.value, 0xFF654321);
await tester.pumpWidget(
MediaQuery(
data: const MediaQueryData(),
child: boilerplate(child: const CupertinoButton(
color: bgColor,
disabledColor: inactive,
onPressed: null,
child: Text('Skeuomorph me'),
)),
),
);
boxDecoration = tester.widget<DecoratedBox>(
find.widgetWithText(DecoratedBox, 'Skeuomorph me'),
).decoration as BoxDecoration;
// Disabled color.
expect(boxDecoration.color!.value, 0xFF111111);
});
testWidgets('Button respects themes', (WidgetTester tester) async {
late TextStyle textStyle;
await tester.pumpWidget(
CupertinoApp(
home: CupertinoButton(
onPressed: () { },
child: Builder(builder: (BuildContext context) {
textStyle = DefaultTextStyle.of(context).style;
return const Placeholder();
}),
),
),
);
expect(textStyle.color, CupertinoColors.activeBlue);
await tester.pumpWidget(
CupertinoApp(
home: CupertinoButton.filled(
onPressed: () { },
child: Builder(builder: (BuildContext context) {
textStyle = DefaultTextStyle.of(context).style;
return const Placeholder();
}),
),
),
);
expect(textStyle.color, isSameColorAs(CupertinoColors.white));
BoxDecoration decoration = tester.widget<DecoratedBox>(
find.descendant(
of: find.byType(CupertinoButton),
matching: find.byType(DecoratedBox),
),
).decoration as BoxDecoration;
expect(decoration.color, CupertinoColors.activeBlue);
await tester.pumpWidget(
CupertinoApp(
theme: const CupertinoThemeData(brightness: Brightness.dark),
home: CupertinoButton(
onPressed: () { },
child: Builder(builder: (BuildContext context) {
textStyle = DefaultTextStyle.of(context).style;
return const Placeholder();
}),
),
),
);
expect(textStyle.color, isSameColorAs(CupertinoColors.systemBlue.darkColor));
await tester.pumpWidget(
CupertinoApp(
theme: const CupertinoThemeData(brightness: Brightness.dark),
home: CupertinoButton.filled(
onPressed: () { },
child: Builder(builder: (BuildContext context) {
textStyle = DefaultTextStyle.of(context).style;
return const Placeholder();
}),
),
),
);
expect(textStyle.color, isSameColorAs(CupertinoColors.black));
decoration = tester.widget<DecoratedBox>(
find.descendant(
of: find.byType(CupertinoButton),
matching: find.byType(DecoratedBox),
),
).decoration as BoxDecoration;
expect(decoration.color, isSameColorAs(CupertinoColors.systemBlue.darkColor));
});
testWidgets('Hovering over Cupertino button updates cursor to clickable on Web', (WidgetTester tester) async {
await tester.pumpWidget(
CupertinoApp(
home: Center(
child: CupertinoButton.filled(
onPressed: () { },
child: const Text('Tap me'),
),
),
),
);
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse, pointer: 1);
await gesture.addPointer(location: const Offset(10, 10));
await tester.pumpAndSettle();
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.basic);
final Offset button = tester.getCenter(find.byType(CupertinoButton));
await gesture.moveTo(button);
await tester.pumpAndSettle();
expect(
RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1),
kIsWeb ? SystemMouseCursors.click : SystemMouseCursors.basic,
);
});
}
Widget boilerplate({ required Widget child }) {
return Directionality(
textDirection: TextDirection.ltr,
child: Center(child: child),
);
}
| flutter/packages/flutter/test/cupertino/button_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/button_test.dart",
"repo_id": "flutter",
"token_count": 6466
} | 679 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
@Tags(<String>['reduced-test-set'])
library;
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
final Offset basicOffset = Offset(CupertinoMagnifier.kDefaultSize.width / 2,
CupertinoMagnifier.kDefaultSize.height - CupertinoMagnifier.kMagnifierAboveFocalPoint);
const Rect reasonableTextField = Rect.fromLTRB(0, 100, 200, 200);
final MagnifierController magnifierController = MagnifierController();
// Make sure that your gesture in magnifierInfo is within the line in magnifierInfo,
// or else the magnifier status will stay hidden and this will not complete.
Future<void> showCupertinoMagnifier(
BuildContext context,
WidgetTester tester,
ValueNotifier<MagnifierInfo> magnifierInfo,
) async {
final Future<void> magnifierShown = magnifierController.show(
context: context,
builder: (_) => CupertinoTextMagnifier(
controller: magnifierController,
magnifierInfo: magnifierInfo,
));
WidgetsBinding.instance.scheduleFrame();
await tester.pumpAndSettle();
await magnifierShown;
}
tearDown(() async {
magnifierController.removeFromOverlay();
});
group('CupertinoTextEditingMagnifier', () {
group('position', () {
Offset getMagnifierPosition(WidgetTester tester) {
final AnimatedPositioned animatedPositioned =
tester.firstWidget(find.byType(AnimatedPositioned));
return Offset(
animatedPositioned.left ?? 0, animatedPositioned.top ?? 0);
}
testWidgets('should be at gesture position if does not violate any positioning rules', (WidgetTester tester) async {
final Key fakeTextFieldKey = UniqueKey();
final Key outerKey = UniqueKey();
await tester.pumpWidget(
ColoredBox(
key: outerKey,
color: const Color.fromARGB(255, 0, 255, 179),
child: MaterialApp(
home: Center(
child: Container(
key: fakeTextFieldKey,
width: 10,
height: 10,
color: Colors.red,
child: const Placeholder(),
),
),
),
),
);
final BuildContext context = tester.element(find.byType(Placeholder));
// Magnifier should be positioned directly over the red square.
final RenderBox tapPointRenderBox =
tester.firstRenderObject(find.byKey(fakeTextFieldKey)) as RenderBox;
final Rect fakeTextFieldRect =
tapPointRenderBox.localToGlobal(Offset.zero) & tapPointRenderBox.size;
final ValueNotifier<MagnifierInfo> magnifier =
ValueNotifier<MagnifierInfo>(
MagnifierInfo(
currentLineBoundaries: fakeTextFieldRect,
fieldBounds: fakeTextFieldRect,
caretRect: fakeTextFieldRect,
// The tap position is dragBelow units below the text field.
globalGesturePosition: fakeTextFieldRect.center,
),
);
addTearDown(magnifier.dispose);
await showCupertinoMagnifier(context, tester, magnifier);
// Should show two red squares; original, and one in the magnifier,
// directly ontop of one another.
await expectLater(
find.byKey(outerKey),
matchesGoldenFile('cupertino_magnifier.position.default.png'),
);
});
testWidgets('should never horizontally be outside of Screen Padding', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
color: Color.fromARGB(7, 0, 129, 90),
home: Placeholder(),
),
);
final BuildContext context = tester.firstElement(find.byType(Placeholder));
final ValueNotifier<MagnifierInfo> magnifierInfo = ValueNotifier<MagnifierInfo>(
MagnifierInfo(
currentLineBoundaries: reasonableTextField,
fieldBounds: reasonableTextField,
caretRect: reasonableTextField,
// The tap position is far out of the right side of the app.
globalGesturePosition:
Offset(MediaQuery.sizeOf(context).width + 100, 0),
),
);
addTearDown(magnifierInfo.dispose);
await showCupertinoMagnifier(
context,
tester,
magnifierInfo,
);
// Should be less than the right edge, since we have padding.
expect(getMagnifierPosition(tester).dx,
lessThan(MediaQuery.sizeOf(context).width));
});
testWidgets('should have some vertical drag', (WidgetTester tester) async {
final double dragPositionBelowTextField = reasonableTextField.center.dy + 30;
await tester.pumpWidget(
const MaterialApp(
color: Color.fromARGB(7, 0, 129, 90),
home: Placeholder(),
),
);
final BuildContext context =
tester.firstElement(find.byType(Placeholder));
final ValueNotifier<MagnifierInfo> magnifierInfo =
ValueNotifier<MagnifierInfo>(
MagnifierInfo(
currentLineBoundaries: reasonableTextField,
fieldBounds: reasonableTextField,
caretRect: reasonableTextField,
// The tap position is dragBelow units below the text field.
globalGesturePosition: Offset(
MediaQuery.sizeOf(context).width / 2,
dragPositionBelowTextField),
),
);
addTearDown(magnifierInfo.dispose);
await showCupertinoMagnifier(
context,
tester,
magnifierInfo,
);
// The magnifier Y should be greater than the text field, since we "dragged" it down.
expect(getMagnifierPosition(tester).dy + basicOffset.dy,
greaterThan(reasonableTextField.center.dy));
expect(getMagnifierPosition(tester).dy + basicOffset.dy,
lessThan(dragPositionBelowTextField));
});
});
group('status', () {
testWidgets('should hide if gesture is far below the text field', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
color: Color.fromARGB(7, 0, 129, 90),
home: Placeholder(),
),
);
final BuildContext context =
tester.firstElement(find.byType(Placeholder));
final ValueNotifier<MagnifierInfo> magnifierInfo =
ValueNotifier<MagnifierInfo>(
MagnifierInfo(
currentLineBoundaries: reasonableTextField,
fieldBounds: reasonableTextField,
caretRect: reasonableTextField,
// The tap position is dragBelow units below the text field.
globalGesturePosition: Offset(
MediaQuery.sizeOf(context).width / 2, reasonableTextField.top),
),
);
addTearDown(magnifierInfo.dispose);
// Show the magnifier initially, so that we get it in a not hidden state.
await showCupertinoMagnifier(context, tester, magnifierInfo);
// Move the gesture to one that should hide it.
magnifierInfo.value = MagnifierInfo(
currentLineBoundaries: reasonableTextField,
fieldBounds: reasonableTextField,
caretRect: reasonableTextField,
globalGesturePosition: magnifierInfo.value.globalGesturePosition + const Offset(0, 100),
);
await tester.pumpAndSettle();
expect(magnifierController.shown, false);
expect(magnifierController.overlayEntry, isNotNull);
});
testWidgets('should re-show if gesture moves back up',
(WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
color: Color.fromARGB(7, 0, 129, 90),
home: Placeholder(),
),
);
final BuildContext context =
tester.firstElement(find.byType(Placeholder));
final ValueNotifier<MagnifierInfo> magnifierInfo =
ValueNotifier<MagnifierInfo>(
MagnifierInfo(
currentLineBoundaries: reasonableTextField,
fieldBounds: reasonableTextField,
caretRect: reasonableTextField,
// The tap position is dragBelow units below the text field.
globalGesturePosition: Offset(MediaQuery.sizeOf(context).width / 2, reasonableTextField.top),
),
);
addTearDown(magnifierInfo.dispose);
// Show the magnifier initially, so that we get it in a not hidden state.
await showCupertinoMagnifier(context, tester, magnifierInfo);
// Move the gesture to one that should hide it.
magnifierInfo.value = MagnifierInfo(
currentLineBoundaries: reasonableTextField,
fieldBounds: reasonableTextField,
caretRect: reasonableTextField,
globalGesturePosition:
magnifierInfo.value.globalGesturePosition + const Offset(0, 100));
await tester.pumpAndSettle();
expect(magnifierController.shown, false);
expect(magnifierController.overlayEntry, isNotNull);
// Return the gesture to one that shows it.
magnifierInfo.value = MagnifierInfo(
currentLineBoundaries: reasonableTextField,
fieldBounds: reasonableTextField,
caretRect: reasonableTextField,
globalGesturePosition: Offset(MediaQuery.sizeOf(context).width / 2,
reasonableTextField.top));
await tester.pumpAndSettle();
expect(magnifierController.shown, true);
expect(magnifierController.overlayEntry, isNotNull);
});
});
});
}
| flutter/packages/flutter/test/cupertino/magnifier_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/magnifier_test.dart",
"repo_id": "flutter",
"token_count": 4195
} | 680 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:collection';
import 'package:flutter/cupertino.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/semantics_tester.dart';
RenderBox getRenderSegmentedControl(WidgetTester tester) {
return tester.allRenderObjects.firstWhere(
(RenderObject currentObject) {
return currentObject.toStringShort().contains('_RenderSegmentedControl');
},
) as RenderBox;
}
Rect currentUnscaledThumbRect(WidgetTester tester, { bool useGlobalCoordinate = false }) {
final dynamic renderSegmentedControl = getRenderSegmentedControl(tester);
// Using dynamic to access private class in test.
// ignore: avoid_dynamic_calls
final Rect local = renderSegmentedControl.currentThumbRect as Rect;
if (!useGlobalCoordinate) {
return local;
}
final RenderBox segmentedControl = renderSegmentedControl as RenderBox;
return local.shift(segmentedControl.localToGlobal(Offset.zero));
}
int? getHighlightedIndex(WidgetTester tester) {
return (getRenderSegmentedControl(tester) as dynamic).highlightedIndex as int?;
}
Color getThumbColor(WidgetTester tester) {
return (getRenderSegmentedControl(tester) as dynamic).thumbColor as Color;
}
double currentThumbScale(WidgetTester tester) {
return (getRenderSegmentedControl(tester) as dynamic).thumbScale as double;
}
Widget setupSimpleSegmentedControl() {
const Map<int, Widget> children = <int, Widget>{
0: Text('Child 1'),
1: Text('Child 2'),
};
return boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
);
}
StateSetter? setState;
int? groupValue = 0;
void defaultCallback(int? newValue) {
setState!(() { groupValue = newValue; });
}
Widget boilerplate({ required WidgetBuilder builder }) {
return Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: StatefulBuilder(builder: (BuildContext context, StateSetter setter) {
setState = setter;
return builder(context);
}),
),
);
}
void main() {
setUp(() {
setState = null;
groupValue = 0;
});
testWidgets('Need at least 2 children', (WidgetTester tester) async {
groupValue = null;
await expectLater(
() => tester.pumpWidget(
CupertinoSlidingSegmentedControl<int>(
children: const <int, Widget>{},
groupValue: groupValue,
onValueChanged: defaultCallback,
),
),
throwsA(isAssertionError.having(
(AssertionError error) => error.toString(),
'.toString()',
contains('children.length'),
)),
);
await expectLater(
() => tester.pumpWidget(
CupertinoSlidingSegmentedControl<int>(
children: const <int, Widget>{0: Text('Child 1')},
groupValue: groupValue,
onValueChanged: defaultCallback,
),
),
throwsA(isAssertionError.having(
(AssertionError error) => error.toString(),
'.toString()',
contains('children.length'),
)),
);
groupValue = -1;
await expectLater(
() => tester.pumpWidget(
CupertinoSlidingSegmentedControl<int>(
children: const <int, Widget>{
0: Text('Child 1'),
1: Text('Child 2'),
2: Text('Child 3'),
},
groupValue: groupValue,
onValueChanged: defaultCallback,
),
),
throwsA(isAssertionError.having(
(AssertionError error) => error.toString(),
'.toString()',
contains('groupValue must be either null or one of the keys in the children map'),
)),
);
});
testWidgets('Padding works', (WidgetTester tester) async {
const Key key = Key('Container');
const Map<int, Widget> children = <int, Widget>{
0: Text('Child 1'),
1: Text('Child 2'),
};
Future<void> verifyPadding({ EdgeInsets? padding }) async {
final EdgeInsets effectivePadding = padding ?? const EdgeInsets.symmetric(vertical: 2, horizontal: 3);
final Rect segmentedControlRect = tester.getRect(find.byKey(key));
expect(
tester.getTopLeft(find.ancestor(of: find.byWidget(children[0]!), matching: find.byType(MetaData))),
segmentedControlRect.topLeft + effectivePadding.topLeft,
);
expect(
tester.getBottomLeft(find.ancestor(of: find.byWidget(children[0]!), matching: find.byType(MetaData))),
segmentedControlRect.bottomLeft + effectivePadding.bottomLeft,
);
expect(
tester.getTopRight(find.ancestor(of: find.byWidget(children[1]!), matching: find.byType(MetaData))),
segmentedControlRect.topRight + effectivePadding.topRight,
);
expect(
tester.getBottomRight(find.ancestor(of: find.byWidget(children[1]!), matching: find.byType(MetaData))),
segmentedControlRect.bottomRight + effectivePadding.bottomRight,
);
}
await tester.pumpWidget(
boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
key: key,
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
),
);
// Default padding works.
await verifyPadding();
// Switch to Child 2 padding should remain the same.
await tester.tap(find.text('Child 2'));
await tester.pumpAndSettle();
await verifyPadding();
await tester.pumpWidget(
boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
key: key,
padding: const EdgeInsets.fromLTRB(1, 3, 5, 7),
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
),
);
// Custom padding works.
await verifyPadding(padding: const EdgeInsets.fromLTRB(1, 3, 5, 7));
// Switch back to Child 1 padding should remain the same.
await tester.tap(find.text('Child 1'));
await tester.pumpAndSettle();
await verifyPadding(padding: const EdgeInsets.fromLTRB(1, 3, 5, 7));
});
testWidgets('Tap changes toggle state', (WidgetTester tester) async {
const Map<int, Widget> children = <int, Widget>{
0: Text('Child 1'),
1: Text('Child 2'),
2: Text('Child 3'),
};
await tester.pumpWidget(
boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
key: const ValueKey<String>('Segmented Control'),
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
),
);
expect(groupValue, 0);
await tester.tap(find.text('Child 2'));
expect(groupValue, 1);
// Tapping the currently selected item should not change groupValue.
await tester.tap(find.text('Child 2'));
expect(groupValue, 1);
});
testWidgets(
'Segmented controls respect theme',
(WidgetTester tester) async {
const Map<int, Widget> children = <int, Widget>{
0: Text('Child 1'),
1: Icon(IconData(1)),
};
await tester.pumpWidget(
CupertinoApp(
theme: const CupertinoThemeData(brightness: Brightness.dark),
home: boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
),
),
);
DefaultTextStyle textStyle = tester.widget(find.widgetWithText(DefaultTextStyle, 'Child 1').first);
expect(textStyle.style.fontWeight, FontWeight.w500);
await tester.tap(find.byIcon(const IconData(1)));
await tester.pump();
await tester.pumpAndSettle();
textStyle = tester.widget(find.widgetWithText(DefaultTextStyle, 'Child 1').first);
expect(groupValue, 1);
expect(textStyle.style.fontWeight, FontWeight.normal);
},
);
testWidgets('SegmentedControl dark mode', (WidgetTester tester) async {
const Map<int, Widget> children = <int, Widget>{
0: Text('Child 1'),
1: Icon(IconData(1)),
};
Brightness brightness = Brightness.light;
late StateSetter setState;
await tester.pumpWidget(
StatefulBuilder(
builder: (BuildContext context, StateSetter setter) {
setState = setter;
return MediaQuery(
data: MediaQueryData(platformBrightness: brightness),
child: boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
thumbColor: CupertinoColors.systemGreen,
backgroundColor: CupertinoColors.systemRed,
);
},
),
);
},
),
);
final BoxDecoration decoration = tester.widget<Container>(find.descendant(
of: find.byType(UnconstrainedBox),
matching: find.byType(Container),
)).decoration! as BoxDecoration;
expect(getThumbColor(tester).value, CupertinoColors.systemGreen.color.value);
expect(decoration.color!.value, CupertinoColors.systemRed.color.value);
setState(() { brightness = Brightness.dark; });
await tester.pump();
final BoxDecoration decorationDark = tester.widget<Container>(find.descendant(
of: find.byType(UnconstrainedBox),
matching: find.byType(Container),
)).decoration! as BoxDecoration;
expect(getThumbColor(tester).value, CupertinoColors.systemGreen.darkColor.value);
expect(decorationDark.color!.value, CupertinoColors.systemRed.darkColor.value);
});
testWidgets(
'Children can be non-Text or Icon widgets (in this case, '
'a Container or Placeholder widget)',
(WidgetTester tester) async {
const Map<int, Widget> children = <int, Widget>{
0: Text('Child 1'),
1: SizedBox(width: 50, height: 50),
2: Placeholder(),
};
await tester.pumpWidget(
boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
),
);
},
);
testWidgets('Passed in value is child initially selected', (WidgetTester tester) async {
await tester.pumpWidget(setupSimpleSegmentedControl());
expect(getHighlightedIndex(tester), 0);
});
testWidgets('Null input for value results in no child initially selected', (WidgetTester tester) async {
const Map<int, Widget> children = <int, Widget>{
0: Text('Child 1'),
1: Text('Child 2'),
};
groupValue = null;
await tester.pumpWidget(
StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
);
},
),
);
expect(getHighlightedIndex(tester), null);
});
testWidgets('Long press not-selected child interactions', (WidgetTester tester) async {
const Map<int, Widget> children = <int, Widget>{
0: Text('Child 1'),
1: Text('Child 2'),
2: Text('Child 3'),
3: Text('Child 4'),
4: Text('Child 5'),
};
// Child 3 is initially selected.
groupValue = 2;
await tester.pumpWidget(
boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
),
);
double getChildOpacityByName(String childName) {
return tester.renderObject<RenderAnimatedOpacity>(
find.ancestor(matching: find.byType(AnimatedOpacity), of: find.text(childName)),
).opacity.value;
}
// Opacity 1 with no interaction.
expect(getChildOpacityByName('Child 1'), 1);
final Offset center = tester.getCenter(find.text('Child 1'));
final TestGesture gesture = await tester.startGesture(center);
await tester.pumpAndSettle();
// Opacity drops to 0.2.
expect(getChildOpacityByName('Child 1'), 0.2);
// Move down slightly, slightly outside of the segmented control.
await gesture.moveBy(const Offset(0, 50));
await tester.pumpAndSettle();
expect(getChildOpacityByName('Child 1'), 0.2);
// Move further down and far away from the segmented control.
await gesture.moveBy(const Offset(0, 200));
await tester.pumpAndSettle();
expect(getChildOpacityByName('Child 1'), 1);
// Move to child 5.
await gesture.moveTo(tester.getCenter(find.text('Child 5')));
await tester.pumpAndSettle();
expect(getChildOpacityByName('Child 1'), 1);
expect(getChildOpacityByName('Child 5'), 0.2);
// Move to child 2.
await gesture.moveTo(tester.getCenter(find.text('Child 2')));
await tester.pumpAndSettle();
expect(getChildOpacityByName('Child 1'), 1);
expect(getChildOpacityByName('Child 5'), 1);
expect(getChildOpacityByName('Child 2'), 0.2);
});
testWidgets('Long press does not change the opacity of currently-selected child', (WidgetTester tester) async {
double getChildOpacityByName(String childName) {
return tester.renderObject<RenderAnimatedOpacity>(
find.ancestor(matching: find.byType(AnimatedOpacity), of: find.text(childName)),
).opacity.value;
}
await tester.pumpWidget(setupSimpleSegmentedControl());
final Offset center = tester.getCenter(find.text('Child 1'));
final TestGesture gesture = await tester.startGesture(center);
await tester.pump();
await tester.pumpAndSettle();
expect(getChildOpacityByName('Child 1'), 1);
// Finish gesture to release resources.
await gesture.up();
await tester.pumpAndSettle();
});
testWidgets('Height of segmented control is determined by tallest widget', (WidgetTester tester) async {
final Map<int, Widget> children = <int, Widget>{
0: Container(constraints: const BoxConstraints.tightFor(height: 100.0)),
1: Container(constraints: const BoxConstraints.tightFor(height: 400.0)),
2: Container(constraints: const BoxConstraints.tightFor(height: 200.0)),
};
await tester.pumpWidget(
boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
key: const ValueKey<String>('Segmented Control'),
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
),
);
final RenderBox buttonBox = tester.renderObject(
find.byKey(const ValueKey<String>('Segmented Control')),
);
expect(
buttonBox.size.height,
400.0 + 2 * 2, // 2 px padding on both sides.
);
});
testWidgets('Width of each segmented control segment is determined by widest widget', (WidgetTester tester) async {
final Map<int, Widget> children = <int, Widget>{
0: Container(constraints: const BoxConstraints.tightFor(width: 50.0)),
1: Container(constraints: const BoxConstraints.tightFor(width: 100.0)),
2: Container(constraints: const BoxConstraints.tightFor(width: 200.0)),
};
await tester.pumpWidget(
boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
key: const ValueKey<String>('Segmented Control'),
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
),
);
final RenderBox segmentedControl = tester.renderObject(
find.byKey(const ValueKey<String>('Segmented Control')),
);
// Subtract the 8.0px for horizontal padding separator. Remaining width should be allocated
// to each child equally.
final double childWidth = (segmentedControl.size.width - 8) / 3;
expect(childWidth, 200.0 + 9.25 * 2);
});
testWidgets('Width is finite in unbounded space', (WidgetTester tester) async {
const Map<int, Widget> children = <int, Widget>{
0: SizedBox(width: 50),
1: SizedBox(width: 70),
};
await tester.pumpWidget(
boilerplate(
builder: (BuildContext context) {
return Row(
children: <Widget>[
CupertinoSlidingSegmentedControl<int>(
key: const ValueKey<String>('Segmented Control'),
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
),
],
);
},
),
);
final RenderBox segmentedControl = tester.renderObject(
find.byKey(const ValueKey<String>('Segmented Control')),
);
expect(
segmentedControl.size.width,
70 * 2 + 9.25 * 4 + 3 * 2 + 1, // 2 children + 4 child padding + 2 outer padding + 1 separator
);
});
testWidgets('Directionality test - RTL should reverse order of widgets', (WidgetTester tester) async {
const Map<int, Widget> children = <int, Widget>{
0: Text('Child 1'),
1: Text('Child 2'),
};
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.rtl,
child: Center(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setter) {
setState = setter;
return CupertinoSlidingSegmentedControl<int>(
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
),
),
),
);
expect(tester.getTopRight(find.text('Child 1')).dx > tester.getTopRight(find.text('Child 2')).dx, isTrue);
});
testWidgets('Correct initial selection and toggling behavior - RTL', (WidgetTester tester) async {
const Map<int, Widget> children = <int, Widget>{
0: Text('Child 1'),
1: Text('Child 2'),
};
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.rtl,
child: Center(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setter) {
setState = setter;
return CupertinoSlidingSegmentedControl<int>(
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
),
),
),
);
// highlightedIndex is 1 instead of 0 because of RTL.
expect(getHighlightedIndex(tester), 1);
await tester.tap(find.text('Child 2'));
await tester.pump();
expect(getHighlightedIndex(tester), 0);
await tester.tap(find.text('Child 2'));
await tester.pump();
expect(getHighlightedIndex(tester), 0);
});
testWidgets('Segmented control semantics', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
const Map<int, Widget> children = <int, Widget>{
0: Text('Child 1'),
1: Text('Child 2'),
};
await tester.pumpWidget(
boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
),
);
expect(
semantics,
hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics.rootChild(
label: 'Child 1',
flags: <SemanticsFlag>[
SemanticsFlag.isButton,
SemanticsFlag.isInMutuallyExclusiveGroup,
SemanticsFlag.isSelected,
],
actions: <SemanticsAction>[
SemanticsAction.tap,
],
),
TestSemantics.rootChild(
label: 'Child 2',
flags: <SemanticsFlag>[
SemanticsFlag.isButton,
SemanticsFlag.isInMutuallyExclusiveGroup,
],
actions: <SemanticsAction>[
SemanticsAction.tap,
],
),
],
),
ignoreId: true,
ignoreRect: true,
ignoreTransform: true,
),
);
await tester.tap(find.text('Child 2'));
await tester.pump();
expect(
semantics,
hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics.rootChild(
label: 'Child 1',
flags: <SemanticsFlag>[
SemanticsFlag.isButton,
SemanticsFlag.isInMutuallyExclusiveGroup,
],
actions: <SemanticsAction>[
SemanticsAction.tap,
],
),
TestSemantics.rootChild(
label: 'Child 2',
flags: <SemanticsFlag>[
SemanticsFlag.isButton,
SemanticsFlag.isInMutuallyExclusiveGroup,
SemanticsFlag.isSelected,
],
actions: <SemanticsAction>[
SemanticsAction.tap,
],
),
],
),
ignoreId: true,
ignoreRect: true,
ignoreTransform: true,
),
);
semantics.dispose();
});
testWidgets('Non-centered taps work on smaller widgets', (WidgetTester tester) async {
final Map<int, Widget> children = <int, Widget>{};
children[0] = const Text('Child 1');
children[1] = const SizedBox();
await tester.pumpWidget(
boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
key: const ValueKey<String>('Segmented Control'),
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
),
);
expect(groupValue, 0);
final Offset centerOfTwo = tester.getCenter(find.byWidget(children[1]!));
// Tap within the bounds of children[1], but not at the center.
// children[1] is a SizedBox thus not hittable by itself.
await tester.tapAt(centerOfTwo + const Offset(10, 0));
expect(groupValue, 1);
});
testWidgets('Hit-tests report accurate local position in segments', (WidgetTester tester) async {
final Map<int, Widget> children = <int, Widget>{};
late TapDownDetails tapDownDetails;
children[0] = GestureDetector(
behavior: HitTestBehavior.opaque,
onTapDown: (TapDownDetails details) { tapDownDetails = details; },
child: const SizedBox(width: 200, height: 200),
);
children[1] = const Text('Child 2');
await tester.pumpWidget(
boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
key: const ValueKey<String>('Segmented Control'),
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
),
);
expect(groupValue, 0);
final Offset segment0GlobalOffset = tester.getTopLeft(find.byWidget(children[0]!));
await tester.tapAt(segment0GlobalOffset + const Offset(7, 11));
expect(tapDownDetails.localPosition, const Offset(7, 11));
expect(tapDownDetails.globalPosition, segment0GlobalOffset + const Offset(7, 11));
});
testWidgets('Thumb animation is correct when the selected segment changes', (WidgetTester tester) async {
await tester.pumpWidget(setupSimpleSegmentedControl());
final Rect initialRect = currentUnscaledThumbRect(tester, useGlobalCoordinate: true);
expect(currentThumbScale(tester), 1);
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.text('Child 2')));
await tester.pump();
// Does not move until tapUp.
expect(currentThumbScale(tester), 1);
expect(currentUnscaledThumbRect(tester, useGlobalCoordinate: true), initialRect);
// Tap up and the sliding animation should play.
await gesture.up();
await tester.pump();
// 10 ms isn't long enough for this gesture to be recognized as a longpress.
await tester.pump(const Duration(milliseconds: 10));
expect(currentThumbScale(tester), 1);
expect(
currentUnscaledThumbRect(tester, useGlobalCoordinate: true).center.dx,
greaterThan(initialRect.center.dx),
);
await tester.pumpAndSettle();
expect(currentThumbScale(tester), 1);
expect(
currentUnscaledThumbRect(tester, useGlobalCoordinate: true).center,
// We're using a critically damped spring so expect the value of the
// animation controller to not be 1.
offsetMoreOrLessEquals(tester.getCenter(find.text('Child 2')), epsilon: 0.01),
);
// Press the currently selected widget.
await gesture.down(tester.getCenter(find.text('Child 2')));
await tester.pump();
await tester.pump(const Duration(milliseconds: 10));
// The thumb shrinks but does not moves towards left.
expect(currentThumbScale(tester), lessThan(1));
expect(
currentUnscaledThumbRect(tester, useGlobalCoordinate: true).center,
offsetMoreOrLessEquals(tester.getCenter(find.text('Child 2')), epsilon: 0.01),
);
await tester.pumpAndSettle();
expect(currentThumbScale(tester), moreOrLessEquals(0.95, epsilon: 0.01));
expect(
currentUnscaledThumbRect(tester, useGlobalCoordinate: true).center,
offsetMoreOrLessEquals(tester.getCenter(find.text('Child 2')), epsilon: 0.01),
);
// Drag to Child 1.
await gesture.moveTo(tester.getCenter(find.text('Child 1')));
await tester.pump();
await tester.pump(const Duration(milliseconds: 10));
// Moved slightly to the left
expect(currentThumbScale(tester), moreOrLessEquals(0.95, epsilon: 0.01));
expect(
currentUnscaledThumbRect(tester, useGlobalCoordinate: true).center.dx,
lessThan(tester.getCenter(find.text('Child 2')).dx),
);
await tester.pumpAndSettle();
expect(currentThumbScale(tester), moreOrLessEquals(0.95, epsilon: 0.01));
expect(
currentUnscaledThumbRect(tester, useGlobalCoordinate: true).center,
offsetMoreOrLessEquals(tester.getCenter(find.text('Child 1')), epsilon: 0.01),
);
await gesture.up();
await tester.pump();
await tester.pump(const Duration(milliseconds: 10));
expect(currentThumbScale(tester), greaterThan(0.95));
await tester.pumpAndSettle();
expect(currentThumbScale(tester), moreOrLessEquals(1, epsilon: 0.01));
});
testWidgets(
'Thumb does not go out of bounds in animation',
(WidgetTester tester) async {
const Map<int, Widget> children = <int, Widget>{
0: Text('Child 1', maxLines: 1),
1: Text('wiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiide Child 2', maxLines: 1),
2: SizedBox(height: 400),
};
await tester.pumpWidget(boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
));
final Rect initialThumbRect = currentUnscaledThumbRect(tester, useGlobalCoordinate: true);
// Starts animating towards 1.
setState!(() { groupValue = 1; });
await tester.pump(const Duration(milliseconds: 10));
const Map<int, Widget> newChildren = <int, Widget>{
0: Text('C1', maxLines: 1),
1: Text('C2', maxLines: 1),
};
// Now let the segments shrink.
await tester.pumpWidget(boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
children: newChildren,
groupValue: 1,
onValueChanged: defaultCallback,
);
},
));
final RenderBox renderSegmentedControl = getRenderSegmentedControl(tester);
final Offset segmentedControlOrigin = renderSegmentedControl.localToGlobal(Offset.zero);
// Expect the segmented control to be much narrower.
expect(segmentedControlOrigin.dx, greaterThan(initialThumbRect.left));
final Rect thumbRect = currentUnscaledThumbRect(tester, useGlobalCoordinate: true);
expect(initialThumbRect.size.height, 400);
expect(thumbRect.size.height, lessThan(100));
// The new thumbRect should fit in the segmentedControl. The -1 and the +1
// are to account for the thumb's vertical EdgeInsets.
expect(segmentedControlOrigin.dx - 1, lessThanOrEqualTo(thumbRect.left));
expect(segmentedControlOrigin.dx + renderSegmentedControl.size.width + 1, greaterThanOrEqualTo(thumbRect.right));
},
);
testWidgets('Transition is triggered while a transition is already occurring', (WidgetTester tester) async {
const Map<int, Widget> children = <int, Widget>{
0: Text('A'),
1: Text('B'),
2: Text('C'),
};
await tester.pumpWidget(
boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
key: const ValueKey<String>('Segmented Control'),
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
),
);
await tester.tap(find.text('B'));
await tester.pump();
await tester.pump();
await tester.pump(const Duration(milliseconds: 40));
// Between A and B.
final Rect initialThumbRect = currentUnscaledThumbRect(tester, useGlobalCoordinate: true);
expect(initialThumbRect.center.dx, greaterThan(tester.getCenter(find.text('A')).dx));
expect(initialThumbRect.center.dx, lessThan(tester.getCenter(find.text('B')).dx));
// While A to B transition is occurring, press on C.
await tester.tap(find.text('C'));
await tester.pump();
await tester.pump(const Duration(milliseconds: 40));
final Rect secondThumbRect = currentUnscaledThumbRect(tester, useGlobalCoordinate: true);
// Between the initial Rect and B.
expect(secondThumbRect.center.dx, greaterThan(initialThumbRect.center.dx));
expect(secondThumbRect.center.dx, lessThan(tester.getCenter(find.text('B')).dx));
await tester.pump(const Duration(milliseconds: 500));
// Eventually moves to C.
expect(
currentUnscaledThumbRect(tester, useGlobalCoordinate: true).center,
offsetMoreOrLessEquals(tester.getCenter(find.text('C')), epsilon: 0.01),
);
});
testWidgets('Insert segment while animation is running', (WidgetTester tester) async {
final Map<int, Widget> children = SplayTreeMap<int, Widget>((int a, int b) => a - b);
children[0] = const Text('A');
children[2] = const Text('C');
children[3] = const Text('D');
await tester.pumpWidget(
boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
key: const ValueKey<String>('Segmented Control'),
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
),
);
await tester.tap(find.text('D'));
await tester.pump();
await tester.pump(const Duration(milliseconds: 40));
children[1] = const Text('B');
await tester.pumpWidget(
boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
key: const ValueKey<String>('Segmented Control'),
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
),
);
await tester.pumpAndSettle();
// Eventually moves to D.
expect(
currentUnscaledThumbRect(tester, useGlobalCoordinate: true).center,
offsetMoreOrLessEquals(tester.getCenter(find.text('D')), epsilon: 0.01),
);
});
testWidgets('change selection programmatically when dragging', (WidgetTester tester) async {
const Map<int, Widget> children = <int, Widget>{
0: Text('A'),
1: Text('B'),
2: Text('C'),
};
bool callbackCalled = false;
void onValueChanged(int? newValue) {
callbackCalled = true;
}
await tester.pumpWidget(
boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
key: const ValueKey<String>('Segmented Control'),
children: children,
groupValue: groupValue,
onValueChanged: onValueChanged,
);
},
),
);
// Start dragging.
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.text('A')));
await tester.pump();
await tester.pump(const Duration(seconds: 1));
// Change selection programmatically.
setState!(() { groupValue = 1; });
await tester.pump();
await tester.pumpAndSettle();
// The ongoing drag gesture should veto the programmatic change.
expect(
currentUnscaledThumbRect(tester, useGlobalCoordinate: true).center,
offsetMoreOrLessEquals(tester.getCenter(find.text('A')), epsilon: 0.01),
);
// Move the pointer to 'B'. The onValueChanged callback will be called but
// since the parent widget thinks we're already at 'B', it will not trigger
// a rebuild for us.
await gesture.moveTo(tester.getCenter(find.text('B')));
await gesture.up();
await tester.pump();
await tester.pumpAndSettle();
expect(
currentUnscaledThumbRect(tester, useGlobalCoordinate: true).center,
offsetMoreOrLessEquals(tester.getCenter(find.text('B')), epsilon: 0.01),
);
expect(callbackCalled, isFalse);
});
testWidgets('Disallow new gesture when dragging', (WidgetTester tester) async {
const Map<int, Widget> children = <int, Widget>{
0: Text('A'),
1: Text('B'),
2: Text('C'),
};
bool callbackCalled = false;
void onValueChanged(int? newValue) {
callbackCalled = true;
}
await tester.pumpWidget(
boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
key: const ValueKey<String>('Segmented Control'),
children: children,
groupValue: groupValue,
onValueChanged: onValueChanged,
);
},
),
);
// Start dragging.
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.text('A')));
await tester.pump();
await tester.pump(const Duration(seconds: 1));
// Tap a different segment.
await tester.tap(find.text('C'));
await tester.pump();
await tester.pumpAndSettle();
expect(
currentUnscaledThumbRect(tester, useGlobalCoordinate: true).center,
offsetMoreOrLessEquals(tester.getCenter(find.text('A')), epsilon: 0.01),
);
// A different drag.
await tester.drag(find.text('A'), const Offset(300, 0));
await tester.pump();
await tester.pumpAndSettle();
expect(
currentUnscaledThumbRect(tester, useGlobalCoordinate: true).center,
offsetMoreOrLessEquals(tester.getCenter(find.text('A')), epsilon: 0.01),
);
await gesture.up();
expect(callbackCalled, isFalse);
});
testWidgets('gesture outlives the widget', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/63338.
const Map<int, Widget> children = <int, Widget>{
0: Text('A'),
1: Text('B'),
2: Text('C'),
};
await tester.pumpWidget(
boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
key: const ValueKey<String>('Segmented Control'),
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
),
);
// Start dragging.
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.text('A')));
await tester.pump();
await tester.pump(const Duration(seconds: 1));
await tester.pumpWidget(const Placeholder());
await gesture.moveBy(const Offset(200, 0));
await tester.pump();
await tester.pump();
await gesture.up();
await tester.pump();
expect(tester.takeException(), isNull);
});
testWidgets('computeDryLayout is pure', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/73362.
const Map<int, Widget> children = <int, Widget>{
0: Text('A'),
1: Text('B'),
2: Text('C'),
};
const Key key = ValueKey<int>(1);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Center(
child: SizedBox(
width: 10,
child: CupertinoSlidingSegmentedControl<int>(
key: key,
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
),
),
),
),
);
final RenderBox renderBox = getRenderSegmentedControl(tester);
final Size size = renderBox.getDryLayout(const BoxConstraints());
expect(size.width, greaterThan(10));
expect(tester.takeException(), isNull);
});
testWidgets('Has consistent size, independent of groupValue', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/62063.
const Map<int, Widget> children = <int, Widget>{
0: Text('A'),
1: Text('BB'),
2: Text('CCCC'),
};
groupValue = null;
await tester.pumpWidget(
boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
key: const ValueKey<String>('Segmented Control'),
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
),
);
final RenderBox renderBox = getRenderSegmentedControl(tester);
final Size size = renderBox.size;
for (final int value in children.keys) {
setState!(() { groupValue = value; });
await tester.pump();
await tester.pumpAndSettle();
expect(renderBox.size, size);
}
});
testWidgets('ScrollView + SlidingSegmentedControl interaction', (WidgetTester tester) async {
const Map<int, Widget> children = <int, Widget>{
0: Text('Child 1'),
1: Text('Child 2'),
};
final ScrollController scrollController = ScrollController();
addTearDown(scrollController.dispose);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: ListView(
controller: scrollController,
children: <Widget>[
const SizedBox(height: 100),
boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
children: children,
groupValue: groupValue,
onValueChanged: defaultCallback,
);
},
),
const SizedBox(height: 1000),
],
),
),
);
// Tapping still works.
await tester.tap(find.text('Child 2'));
await tester.pump();
expect(groupValue, 1);
// Vertical drag works for the scroll view.
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.text('Child 1')));
// The first moveBy doesn't actually move the scrollable. It's there to make
// sure VerticalDragGestureRecognizer wins the arena. This is due to
// startBehavior being set to DragStartBehavior.start.
await gesture.moveBy(const Offset(0, -100));
await gesture.moveBy(const Offset(0, -100));
await tester.pump();
expect(scrollController.offset, 100);
// Does not affect the segmented control.
expect(groupValue, 1);
await gesture.moveBy(const Offset(0, 100));
await gesture.up();
await tester.pump();
expect(scrollController.offset, 0);
expect(groupValue, 1);
// Long press vertical drag is recognized by the segmented control.
await gesture.down(tester.getCenter(find.text('Child 1')));
await tester.pump(const Duration(milliseconds: 600));
await gesture.moveBy(const Offset(0, -100));
await gesture.moveBy(const Offset(0, -100));
await tester.pump();
// Should not scroll.
expect(scrollController.offset, 0);
expect(groupValue, 1);
await gesture.moveBy(const Offset(0, 100));
await gesture.moveBy(const Offset(0, 100));
await gesture.up();
await tester.pump();
expect(scrollController.offset, 0);
expect(groupValue, 0);
// Horizontal drag is recognized by the segmentedControl.
await gesture.down(tester.getCenter(find.text('Child 1')));
await gesture.moveBy(const Offset(50, 0));
await gesture.moveTo(tester.getCenter(find.text('Child 2')));
await gesture.up();
await tester.pump();
expect(scrollController.offset, 0);
expect(groupValue, 1);
});
testWidgets('Hovering over Cupertino sliding segmented control updates cursor to clickable on Web', (WidgetTester tester) async {
const Map<int, Widget> children = <int, Widget>{
0: Text('A'),
1: Text('BB'),
2: Text('CCCC'),
};
await tester.pumpWidget(
boilerplate(
builder: (BuildContext context) {
return CupertinoSlidingSegmentedControl<int>(
key: const ValueKey<String>('Segmented Control'),
children: children,
onValueChanged: defaultCallback,
);
},
),
);
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse, pointer: 1);
await gesture.addPointer(location: const Offset(10, 10));
await tester.pumpAndSettle();
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.basic);
final Offset firstChild = tester.getCenter(find.text('A'));
await gesture.moveTo(firstChild);
await tester.pumpAndSettle();
expect(
RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1),
kIsWeb ? SystemMouseCursors.click : SystemMouseCursors.basic,
);
});
}
| flutter/packages/flutter/test/cupertino/sliding_segmented_control_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/cupertino/sliding_segmented_control_test.dart",
"repo_id": "flutter",
"token_count": 17834
} | 681 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:io';
import 'package:flutter/foundation.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:leak_tracker_flutter_testing/leak_tracker_flutter_testing.dart';
import '_goldens_io.dart' if (dart.library.html) '_goldens_web.dart'
as flutter_goldens;
/// If true, leak tracking is enabled for all `testWidgets`.
///
/// By default it is false.
/// To enable the leak tracking, either pass the compilation flag
/// `--dart-define LEAK_TRACKING=true` or invoke `export LEAK_TRACKING=true`.
///
/// See documentation for [testWidgets] on how to except individual tests.
bool _isLeakTrackingEnabled() {
if (kIsWeb) {
return false;
}
// The values can be different, one is compile time, another is run time.
return const bool.fromEnvironment('LEAK_TRACKING') ||
(bool.tryParse(Platform.environment['LEAK_TRACKING'] ?? '') ?? false);
}
/// Test configuration for each test library in this directory.
///
/// See https://api.flutter.dev/flutter/flutter_test/flutter_test-library.html.
Future<void> testExecutable(FutureOr<void> Function() testMain) {
// Enable checks because there are many implementations of [RenderBox] in this
// package can benefit from the additional validations.
debugCheckIntrinsicSizes = true;
// Make tap() et al fail if the given finder specifies a widget that would not
// receive the event.
WidgetController.hitTestWarningShouldBeFatal = true;
if (_isLeakTrackingEnabled()) {
LeakTesting.enable();
LeakTracking.warnForUnsupportedPlatforms = false;
LeakTesting.settings = LeakTesting.settings.withIgnored(
createdByTestHelpers: true,
allNotGCed: true,
);
}
// Enable golden file testing using Skia Gold.
return flutter_goldens.testExecutable(testMain);
}
| flutter/packages/flutter/test/flutter_test_config.dart/0 | {
"file_path": "flutter/packages/flutter/test/flutter_test_config.dart",
"repo_id": "flutter",
"token_count": 641
} | 682 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('isWeb is false for flutter tester', () {
expect(kIsWeb, false);
}, skip: kIsWeb); // [intended] kIsWeb is what we are testing here.
}
| flutter/packages/flutter/test/foundation/constants_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/foundation/constants_test.dart",
"repo_id": "flutter",
"token_count": 133
} | 683 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:convert';
import 'dart:ui' as ui;
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
class TestServiceExtensionsBinding extends BindingBase
with SchedulerBinding,
ServicesBinding,
GestureBinding,
PaintingBinding,
SemanticsBinding,
RendererBinding,
WidgetsBinding,
TestDefaultBinaryMessengerBinding {
final Map<String, ServiceExtensionCallback> extensions = <String, ServiceExtensionCallback>{};
final Map<String, List<Map<String, dynamic>>> eventsDispatched = <String, List<Map<String, dynamic>>>{};
@override
@protected
void registerServiceExtension({
required String name,
required ServiceExtensionCallback callback,
}) {
expect(extensions.containsKey(name), isFalse);
extensions[name] = callback;
}
@override
void postEvent(String eventKind, Map<String, dynamic> eventData) {
getEventsDispatched(eventKind).add(eventData);
}
List<Map<String, dynamic>> getEventsDispatched(String eventKind) {
return eventsDispatched.putIfAbsent(eventKind, () => <Map<String, dynamic>>[]);
}
Iterable<Map<String, dynamic>> getServiceExtensionStateChangedEvents(String extensionName) {
return getEventsDispatched('Flutter.ServiceExtensionStateChanged')
.where((Map<String, dynamic> event) => event['extension'] == extensionName);
}
Future<Map<String, dynamic>> testExtension(String name, Map<String, String> arguments) {
expect(extensions.containsKey(name), isTrue);
return extensions[name]!(arguments);
}
int reassembled = 0;
bool pendingReassemble = false;
@override
Future<void> performReassemble() {
reassembled += 1;
pendingReassemble = true;
return super.performReassemble();
}
bool frameScheduled = false;
@override
void scheduleFrame() {
ensureFrameCallbacksRegistered();
frameScheduled = true;
}
Future<void> doFrame() async {
frameScheduled = false;
binding.platformDispatcher.onBeginFrame?.call(Duration.zero);
await flushMicrotasks();
binding.platformDispatcher.onDrawFrame?.call();
binding.platformDispatcher.onReportTimings?.call(<ui.FrameTiming>[]);
}
@override
void scheduleForcedFrame() {
expect(true, isFalse);
}
@override
void scheduleWarmUpFrame() {
expect(pendingReassemble, isTrue);
pendingReassemble = false;
}
Future<void> flushMicrotasks() {
final Completer<void> completer = Completer<void>();
Timer.run(completer.complete);
return completer.future;
}
}
late TestServiceExtensionsBinding binding;
Future<Map<String, dynamic>> hasReassemble(Future<Map<String, dynamic>> pendingResult) async {
bool completed = false;
pendingResult.whenComplete(() { completed = true; });
expect(binding.frameScheduled, isFalse);
await binding.flushMicrotasks();
expect(binding.frameScheduled, isTrue);
expect(completed, isFalse);
await binding.flushMicrotasks();
await binding.doFrame();
await binding.flushMicrotasks();
expect(completed, isTrue);
expect(binding.frameScheduled, isFalse);
return pendingResult;
}
void main() {
final Set<String> testedExtensions = <String>{}; // Add the name of an extension to this set in the test where it is tested.
final List<String?> console = <String?>[];
late PipelineOwner owner;
setUpAll(() async {
binding = TestServiceExtensionsBinding();
final RenderView view = RenderView(view: binding.platformDispatcher.views.single);
owner = PipelineOwner(onSemanticsUpdate: (ui.SemanticsUpdate _) { })
..rootNode = view;
binding.rootPipelineOwner.adoptChild(owner);
binding.addRenderView(view);
view.prepareInitialFrame();
binding.scheduleFrame();
expect(binding.frameScheduled, isTrue);
// We need to test this service extension here because the result is true
// after the first binding.doFrame() call.
Map<String, dynamic> firstFrameResult;
expect(binding.debugDidSendFirstFrameEvent, isFalse);
firstFrameResult = await binding.testExtension(WidgetsServiceExtensions.didSendFirstFrameEvent.name, <String, String>{});
expect(firstFrameResult, <String, String>{'enabled': 'false'});
expect(binding.firstFrameRasterized, isFalse);
firstFrameResult = await binding.testExtension(WidgetsServiceExtensions.didSendFirstFrameRasterizedEvent.name, <String, String>{});
expect(firstFrameResult, <String, String>{'enabled': 'false'});
await binding.doFrame();
expect(binding.debugDidSendFirstFrameEvent, isTrue);
firstFrameResult = await binding.testExtension(WidgetsServiceExtensions.didSendFirstFrameEvent.name, <String, String>{});
expect(firstFrameResult, <String, String>{'enabled': 'true'});
expect(binding.firstFrameRasterized, isTrue);
firstFrameResult = await binding.testExtension(WidgetsServiceExtensions.didSendFirstFrameRasterizedEvent.name, <String, String>{});
expect(firstFrameResult, <String, String>{'enabled': 'true'});
expect(binding.frameScheduled, isFalse);
testedExtensions.add(WidgetsServiceExtensions.didSendFirstFrameEvent.name);
testedExtensions.add(WidgetsServiceExtensions.didSendFirstFrameRasterizedEvent.name);
expect(debugPrint, equals(debugPrintThrottled));
debugPrint = (String? message, { int? wrapWidth }) {
console.add(message);
};
});
tearDownAll(() async {
// See widget_inspector_test.dart for tests of the ext.flutter.inspector
// service extensions included in this count.
int widgetInspectorExtensionCount = 28;
if (WidgetInspectorService.instance.isWidgetCreationTracked()) {
// Some inspector extensions are only exposed if widget creation locations
// are tracked.
widgetInspectorExtensionCount += 2;
}
expect(binding.extensions.keys.where((String name) => name.startsWith('inspector.')), hasLength(widgetInspectorExtensionCount));
// The following service extensions are disabled in web:
// 1. exit
// 2. showPerformanceOverlay
const int disabledExtensions = kIsWeb ? 2 : 0;
// The expected number of registered service extensions in the Flutter
// framework, excluding any that are for the widget inspector (see
// widget_inspector_test.dart for tests of the ext.flutter.inspector service
// extensions). Any test counted here must be tested in this file!
const int serviceExtensionCount = 30;
expect(binding.extensions.length, serviceExtensionCount + widgetInspectorExtensionCount - disabledExtensions);
expect(testedExtensions, hasLength(serviceExtensionCount));
expect(console, isEmpty);
debugPrint = debugPrintThrottled;
binding.rootPipelineOwner.dropChild(owner);
owner
..rootNode = null
..dispose();
});
// The following list is alphabetical, one test per extension.
test('Service extensions - debugAllowBanner', () async {
Map<String, dynamic> result;
expect(binding.frameScheduled, isFalse);
expect(WidgetsApp.debugAllowBannerOverride, true);
result = await binding.testExtension(WidgetsServiceExtensions.debugAllowBanner.name, <String, String>{});
expect(result, <String, String>{'enabled': 'true'});
expect(WidgetsApp.debugAllowBannerOverride, true);
result = await binding.testExtension(WidgetsServiceExtensions.debugAllowBanner.name, <String, String>{'enabled': 'false'});
expect(result, <String, String>{'enabled': 'false'});
expect(WidgetsApp.debugAllowBannerOverride, false);
result = await binding.testExtension(WidgetsServiceExtensions.debugAllowBanner.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(WidgetsApp.debugAllowBannerOverride, false);
result = await binding.testExtension(WidgetsServiceExtensions.debugAllowBanner.name, <String, String>{'enabled': 'true'});
expect(result, <String, String>{'enabled': 'true'});
expect(WidgetsApp.debugAllowBannerOverride, true);
result = await binding.testExtension(WidgetsServiceExtensions.debugAllowBanner.name, <String, String>{});
expect(result, <String, String>{'enabled': 'true'});
expect(WidgetsApp.debugAllowBannerOverride, true);
expect(binding.frameScheduled, isFalse);
testedExtensions.add(WidgetsServiceExtensions.debugAllowBanner.name);
});
test('Service extensions - debugDumpApp', () async {
final Map<String, dynamic> result = await binding.testExtension(WidgetsServiceExtensions.debugDumpApp.name, <String, String>{});
expect(result, <String, dynamic>{
'data': matches('TestServiceExtensionsBinding - DEBUG MODE\n<no tree currently mounted>'),
});
testedExtensions.add(WidgetsServiceExtensions.debugDumpApp.name);
});
test('Service extensions - debugDumpFocusTree', () async {
final Map<String, dynamic> result = await binding.testExtension(WidgetsServiceExtensions.debugDumpFocusTree.name, <String, String>{});
expect(result, <String, dynamic>{
'data': matches(
r'^'
r'FocusManager#[0-9a-f]{5}\n'
r' └─rootScope: FocusScopeNode#[0-9a-f]{5}\(Root Focus Scope\)\n'
r'$',
),
});
testedExtensions.add(WidgetsServiceExtensions.debugDumpFocusTree.name);
});
test('Service extensions - debugDumpRenderTree', () async {
await binding.doFrame();
final Map<String, dynamic> result = await binding.testExtension(RenderingServiceExtensions.debugDumpRenderTree.name, <String, String>{});
expect(result, <String, dynamic>{
'data': matches(
r'^'
r'RenderView#[0-9a-f]{5}\n'
r' debug mode enabled - [a-zA-Z]+\n'
r' view size: Size\(2400\.0, 1800\.0\) \(in physical pixels\)\n'
r' device pixel ratio: 3\.0 \(physical pixels per logical pixel\)\n'
r' configuration: BoxConstraints\(w=800\.0, h=600\.0\) at 3\.0x \(in\n'
r' logical pixels\)\n'
r'$',
),
});
testedExtensions.add(RenderingServiceExtensions.debugDumpRenderTree.name);
});
test('Service extensions - debugDumpLayerTree', () async {
await binding.doFrame();
final Map<String, dynamic> result = await binding.testExtension(RenderingServiceExtensions.debugDumpLayerTree.name, <String, String>{});
expect(result, <String, dynamic>{
'data': matches(
r'^'
r'TransformLayer#[0-9a-f]{5}\n'
r' owner: RenderView#[0-9a-f]{5}\n'
r' creator: RenderView\n'
r' engine layer: (TransformEngineLayer|PersistedTransform)#[0-9a-f]{5}\n'
r' handles: 1\n'
r' offset: Offset\(0\.0, 0\.0\)\n'
r' transform:\n'
r' \[0] 3\.0,0\.0,0\.0,0\.0\n'
r' \[1] 0\.0,3\.0,0\.0,0\.0\n'
r' \[2] 0\.0,0\.0,1\.0,0\.0\n'
r' \[3] 0\.0,0\.0,0\.0,1\.0\n'
r'$',
),
});
testedExtensions.add(RenderingServiceExtensions.debugDumpLayerTree.name);
});
test('Service extensions - debugDumpSemanticsTreeInTraversalOrder', () async {
await binding.doFrame();
final Map<String, dynamic> result = await binding.testExtension(RenderingServiceExtensions.debugDumpSemanticsTreeInTraversalOrder.name, <String, String>{});
expect(result, <String, Object>{
'data': matches(
r'Semantics not generated for RenderView#[0-9a-f]{5}\.\n'
r'For performance reasons, the framework only generates semantics when asked to do so by the platform.\n'
r'Usually, platforms only ask for semantics when assistive technologies \(like screen readers\) are running.\n'
r'To generate semantics, try turning on an assistive technology \(like VoiceOver or TalkBack\) on your device.'
)
});
testedExtensions.add(RenderingServiceExtensions.debugDumpSemanticsTreeInTraversalOrder.name);
});
test('Service extensions - debugDumpSemanticsTreeInInverseHitTestOrder', () async {
await binding.doFrame();
final Map<String, dynamic> result = await binding.testExtension(RenderingServiceExtensions.debugDumpSemanticsTreeInInverseHitTestOrder.name, <String, String>{});
expect(result, <String, Object>{
'data': matches(
r'Semantics not generated for RenderView#[0-9a-f]{5}\.\n'
r'For performance reasons, the framework only generates semantics when asked to do so by the platform.\n'
r'Usually, platforms only ask for semantics when assistive technologies \(like screen readers\) are running.\n'
r'To generate semantics, try turning on an assistive technology \(like VoiceOver or TalkBack\) on your device.'
)
});
testedExtensions.add(RenderingServiceExtensions.debugDumpSemanticsTreeInInverseHitTestOrder.name);
});
test('Service extensions - debugPaint', () async {
final Iterable<Map<String, dynamic>> extensionChangedEvents = binding.getServiceExtensionStateChangedEvents('ext.flutter.${RenderingServiceExtensions.debugPaint.name}');
Map<String, dynamic> extensionChangedEvent;
Map<String, dynamic> result;
Future<Map<String, dynamic>> pendingResult;
bool completed;
expect(binding.frameScheduled, isFalse);
expect(debugPaintSizeEnabled, false);
result = await binding.testExtension(RenderingServiceExtensions.debugPaint.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugPaintSizeEnabled, false);
expect(extensionChangedEvents, isEmpty);
expect(binding.frameScheduled, isFalse);
pendingResult = binding.testExtension(RenderingServiceExtensions.debugPaint.name, <String, String>{'enabled': 'true'});
completed = false;
pendingResult.whenComplete(() { completed = true; });
await binding.flushMicrotasks();
expect(binding.frameScheduled, isTrue);
expect(completed, isFalse);
await binding.doFrame();
await binding.flushMicrotasks();
expect(completed, isTrue);
expect(binding.frameScheduled, isFalse);
result = await pendingResult;
expect(result, <String, String>{'enabled': 'true'});
expect(debugPaintSizeEnabled, true);
expect(extensionChangedEvents.length, 1);
extensionChangedEvent = extensionChangedEvents.last;
expect(extensionChangedEvent['extension'], 'ext.flutter.debugPaint');
expect(extensionChangedEvent['value'], 'true');
result = await binding.testExtension(RenderingServiceExtensions.debugPaint.name, <String, String>{});
expect(result, <String, String>{'enabled': 'true'});
expect(debugPaintSizeEnabled, true);
expect(extensionChangedEvents.length, 1);
expect(binding.frameScheduled, isFalse);
pendingResult = binding.testExtension(RenderingServiceExtensions.debugPaint.name, <String, String>{'enabled': 'false'});
await binding.flushMicrotasks();
expect(binding.frameScheduled, isTrue);
await binding.doFrame();
expect(binding.frameScheduled, isFalse);
result = await pendingResult;
expect(result, <String, String>{'enabled': 'false'});
expect(debugPaintSizeEnabled, false);
expect(extensionChangedEvents.length, 2);
extensionChangedEvent = extensionChangedEvents.last;
expect(extensionChangedEvent['extension'], 'ext.flutter.debugPaint');
expect(extensionChangedEvent['value'], 'false');
result = await binding.testExtension(RenderingServiceExtensions.debugPaint.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugPaintSizeEnabled, false);
expect(extensionChangedEvents.length, 2);
expect(binding.frameScheduled, isFalse);
testedExtensions.add(RenderingServiceExtensions.debugPaint.name);
});
test('Service extensions - debugPaintBaselinesEnabled', () async {
Map<String, dynamic> result;
Future<Map<String, dynamic>> pendingResult;
bool completed;
expect(binding.frameScheduled, isFalse);
expect(debugPaintBaselinesEnabled, false);
result = await binding.testExtension(RenderingServiceExtensions.debugPaintBaselinesEnabled.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugPaintBaselinesEnabled, false);
expect(binding.frameScheduled, isFalse);
pendingResult = binding.testExtension(RenderingServiceExtensions.debugPaintBaselinesEnabled.name, <String, String>{'enabled': 'true'});
completed = false;
pendingResult.whenComplete(() { completed = true; });
await binding.flushMicrotasks();
expect(binding.frameScheduled, isTrue);
expect(completed, isFalse);
await binding.doFrame();
await binding.flushMicrotasks();
expect(completed, isTrue);
expect(binding.frameScheduled, isFalse);
result = await pendingResult;
expect(result, <String, String>{'enabled': 'true'});
expect(debugPaintBaselinesEnabled, true);
result = await binding.testExtension(RenderingServiceExtensions.debugPaintBaselinesEnabled.name, <String, String>{});
expect(result, <String, String>{'enabled': 'true'});
expect(debugPaintBaselinesEnabled, true);
expect(binding.frameScheduled, isFalse);
pendingResult = binding.testExtension(RenderingServiceExtensions.debugPaintBaselinesEnabled.name, <String, String>{'enabled': 'false'});
await binding.flushMicrotasks();
expect(binding.frameScheduled, isTrue);
await binding.doFrame();
expect(binding.frameScheduled, isFalse);
result = await pendingResult;
expect(result, <String, String>{'enabled': 'false'});
expect(debugPaintBaselinesEnabled, false);
result = await binding.testExtension(RenderingServiceExtensions.debugPaintBaselinesEnabled.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugPaintBaselinesEnabled, false);
expect(binding.frameScheduled, isFalse);
testedExtensions.add(RenderingServiceExtensions.debugPaintBaselinesEnabled.name);
});
test('Service extensions - invertOversizedImages', () async {
Map<String, dynamic> result;
Future<Map<String, dynamic>> pendingResult;
bool completed;
expect(binding.frameScheduled, isFalse);
expect(debugInvertOversizedImages, false);
result = await binding.testExtension(RenderingServiceExtensions.invertOversizedImages.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugInvertOversizedImages, false);
expect(binding.frameScheduled, isFalse);
pendingResult = binding.testExtension(RenderingServiceExtensions.invertOversizedImages.name, <String, String>{'enabled': 'true'});
completed = false;
pendingResult.whenComplete(() { completed = true; });
await binding.flushMicrotasks();
expect(binding.frameScheduled, isTrue);
expect(completed, isFalse);
await binding.doFrame();
await binding.flushMicrotasks();
expect(completed, isTrue);
expect(binding.frameScheduled, isFalse);
result = await pendingResult;
expect(result, <String, String>{'enabled': 'true'});
expect(debugInvertOversizedImages, true);
result = await binding.testExtension(RenderingServiceExtensions.invertOversizedImages.name, <String, String>{});
expect(result, <String, String>{'enabled': 'true'});
expect(debugInvertOversizedImages, true);
expect(binding.frameScheduled, isFalse);
pendingResult = binding.testExtension(RenderingServiceExtensions.invertOversizedImages.name, <String, String>{'enabled': 'false'});
await binding.flushMicrotasks();
expect(binding.frameScheduled, isTrue);
await binding.doFrame();
expect(binding.frameScheduled, isFalse);
result = await pendingResult;
expect(result, <String, String>{'enabled': 'false'});
expect(debugInvertOversizedImages, false);
result = await binding.testExtension(RenderingServiceExtensions.invertOversizedImages.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugInvertOversizedImages, false);
expect(binding.frameScheduled, isFalse);
testedExtensions.add(RenderingServiceExtensions.invertOversizedImages.name);
});
test('Service extensions - profileWidgetBuilds', () async {
Map<String, dynamic> result;
expect(binding.frameScheduled, isFalse);
expect(debugProfileBuildsEnabled, false);
result = await binding.testExtension(WidgetsServiceExtensions.profileWidgetBuilds.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugProfileBuildsEnabled, false);
result = await binding.testExtension(WidgetsServiceExtensions.profileWidgetBuilds.name, <String, String>{'enabled': 'true'});
expect(result, <String, String>{'enabled': 'true'});
expect(debugProfileBuildsEnabled, true);
result = await binding.testExtension(WidgetsServiceExtensions.profileWidgetBuilds.name, <String, String>{});
expect(result, <String, String>{'enabled': 'true'});
expect(debugProfileBuildsEnabled, true);
result = await binding.testExtension(WidgetsServiceExtensions.profileWidgetBuilds.name, <String, String>{'enabled': 'false'});
expect(result, <String, String>{'enabled': 'false'});
expect(debugProfileBuildsEnabled, false);
result = await binding.testExtension(WidgetsServiceExtensions.profileWidgetBuilds.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugProfileBuildsEnabled, false);
expect(binding.frameScheduled, isFalse);
testedExtensions.add(WidgetsServiceExtensions.profileWidgetBuilds.name);
});
test('Service extensions - profileUserWidgetBuilds', () async {
Map<String, dynamic> result;
expect(binding.frameScheduled, isFalse);
expect(debugProfileBuildsEnabledUserWidgets, false);
result = await binding.testExtension(WidgetsServiceExtensions.profileUserWidgetBuilds.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugProfileBuildsEnabledUserWidgets, false);
result = await binding.testExtension(WidgetsServiceExtensions.profileUserWidgetBuilds.name, <String, String>{'enabled': 'true'});
expect(result, <String, String>{'enabled': 'true'});
expect(debugProfileBuildsEnabledUserWidgets, true);
result = await binding.testExtension(WidgetsServiceExtensions.profileUserWidgetBuilds.name, <String, String>{});
expect(result, <String, String>{'enabled': 'true'});
expect(debugProfileBuildsEnabledUserWidgets, true);
result = await binding.testExtension(WidgetsServiceExtensions.profileUserWidgetBuilds.name, <String, String>{'enabled': 'false'});
expect(result, <String, String>{'enabled': 'false'});
expect(debugProfileBuildsEnabledUserWidgets, false);
result = await binding.testExtension(WidgetsServiceExtensions.profileUserWidgetBuilds.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugProfileBuildsEnabledUserWidgets, false);
expect(binding.frameScheduled, isFalse);
testedExtensions.add(WidgetsServiceExtensions.profileUserWidgetBuilds.name);
});
test('Service extensions - profileRenderObjectPaints', () async {
Map<String, dynamic> result;
expect(binding.frameScheduled, isFalse);
expect(debugProfileBuildsEnabled, false);
result = await binding.testExtension(RenderingServiceExtensions.profileRenderObjectPaints.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugProfilePaintsEnabled, false);
result = await binding.testExtension(RenderingServiceExtensions.profileRenderObjectPaints.name, <String, String>{'enabled': 'true'});
expect(result, <String, String>{'enabled': 'true'});
expect(debugProfilePaintsEnabled, true);
result = await binding.testExtension(RenderingServiceExtensions.profileRenderObjectPaints.name, <String, String>{});
expect(result, <String, String>{'enabled': 'true'});
expect(debugProfilePaintsEnabled, true);
result = await binding.testExtension(RenderingServiceExtensions.profileRenderObjectPaints.name, <String, String>{'enabled': 'false'});
expect(result, <String, String>{'enabled': 'false'});
expect(debugProfilePaintsEnabled, false);
result = await binding.testExtension(RenderingServiceExtensions.profileRenderObjectPaints.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugProfilePaintsEnabled, false);
expect(binding.frameScheduled, isFalse);
testedExtensions.add(RenderingServiceExtensions.profileRenderObjectPaints.name);
});
test('Service extensions - profileRenderObjectLayouts', () async {
Map<String, dynamic> result;
expect(binding.frameScheduled, isFalse);
expect(debugProfileLayoutsEnabled, false);
result = await binding.testExtension(RenderingServiceExtensions.profileRenderObjectLayouts.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugProfileLayoutsEnabled, false);
result = await binding.testExtension(RenderingServiceExtensions.profileRenderObjectLayouts.name, <String, String>{'enabled': 'true'});
expect(result, <String, String>{'enabled': 'true'});
expect(debugProfileLayoutsEnabled, true);
result = await binding.testExtension(RenderingServiceExtensions.profileRenderObjectLayouts.name, <String, String>{});
expect(result, <String, String>{'enabled': 'true'});
expect(debugProfileLayoutsEnabled, true);
result = await binding.testExtension(RenderingServiceExtensions.profileRenderObjectLayouts.name, <String, String>{'enabled': 'false'});
expect(result, <String, String>{'enabled': 'false'});
expect(debugProfileLayoutsEnabled, false);
result = await binding.testExtension(RenderingServiceExtensions.profileRenderObjectLayouts.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugProfileLayoutsEnabled, false);
expect(binding.frameScheduled, isFalse);
testedExtensions.add(RenderingServiceExtensions.profileRenderObjectLayouts.name);
});
test('Service extensions - profilePlatformChannels', () async {
Map<String, dynamic> result;
expect(debugProfilePlatformChannels, false);
result = await binding.testExtension(ServicesServiceExtensions.profilePlatformChannels.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugProfilePlatformChannels, false);
result = await binding.testExtension(ServicesServiceExtensions.profilePlatformChannels.name, <String, String>{'enabled': 'true'});
expect(result, <String, String>{'enabled': 'true'});
expect(debugProfilePlatformChannels, true);
result = await binding.testExtension(ServicesServiceExtensions.profilePlatformChannels.name, <String, String>{});
expect(result, <String, String>{'enabled': 'true'});
expect(debugProfilePlatformChannels, true);
result = await binding.testExtension(ServicesServiceExtensions.profilePlatformChannels.name, <String, String>{'enabled': 'false'});
expect(result, <String, String>{'enabled': 'false'});
expect(debugProfilePlatformChannels, false);
result = await binding.testExtension(ServicesServiceExtensions.profilePlatformChannels.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugProfilePlatformChannels, false);
testedExtensions.add(ServicesServiceExtensions.profilePlatformChannels.name);
});
test('Service extensions - evict', () async {
Map<String, dynamic> result;
bool completed;
completed = false;
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger.setMockMessageHandler('flutter/assets', (ByteData? message) async {
expect(utf8.decode(message!.buffer.asUint8List()), 'test');
completed = true;
return ByteData(5); // 0x0000000000
});
bool data;
data = await rootBundle.loadStructuredData<bool>('test', (String value) async {
expect(value, '\x00\x00\x00\x00\x00');
return true;
});
expect(data, isTrue);
expect(completed, isTrue);
completed = false;
data = await rootBundle.loadStructuredData('test', (String value) async {
throw Error();
});
expect(data, isTrue);
expect(completed, isFalse);
result = await binding.testExtension(ServicesServiceExtensions.evict.name, <String, String>{'value': 'test'});
expect(result, <String, String>{'value': ''});
expect(completed, isFalse);
data = await rootBundle.loadStructuredData<bool>('test', (String value) async {
expect(value, '\x00\x00\x00\x00\x00');
return false;
});
expect(data, isFalse);
expect(completed, isTrue);
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger.setMockMessageHandler('flutter/assets', null);
testedExtensions.add(ServicesServiceExtensions.evict.name);
});
test('Service extensions - exit', () async {
// no test for _calling_ 'exit', because that should terminate the process!
// Not expecting extension to be available for web platform.
expect(binding.extensions.containsKey(FoundationServiceExtensions.exit.name), !isBrowser);
testedExtensions.add(FoundationServiceExtensions.exit.name);
});
test('Service extensions - platformOverride', () async {
final Iterable<Map<String, dynamic>> extensionChangedEvents = binding.getServiceExtensionStateChangedEvents('ext.flutter.platformOverride');
Map<String, dynamic> extensionChangedEvent;
Map<String, dynamic> result;
expect(binding.reassembled, 0);
expect(defaultTargetPlatform, TargetPlatform.android);
result = await binding.testExtension(FoundationServiceExtensions.platformOverride.name, <String, String>{});
expect(result, <String, String>{'value': 'android'});
expect(defaultTargetPlatform, TargetPlatform.android);
expect(extensionChangedEvents, isEmpty);
result = await hasReassemble(binding.testExtension(FoundationServiceExtensions.platformOverride.name, <String, String>{'value': 'iOS'}));
expect(result, <String, String>{'value': 'iOS'});
expect(binding.reassembled, 1);
expect(defaultTargetPlatform, TargetPlatform.iOS);
expect(extensionChangedEvents.length, 1);
extensionChangedEvent = extensionChangedEvents.last;
expect(extensionChangedEvent['extension'], 'ext.flutter.platformOverride');
expect(extensionChangedEvent['value'], 'iOS');
result = await hasReassemble(binding.testExtension(FoundationServiceExtensions.platformOverride.name, <String, String>{'value': 'macOS'}));
expect(result, <String, String>{'value': 'macOS'});
expect(binding.reassembled, 2);
expect(defaultTargetPlatform, TargetPlatform.macOS);
expect(extensionChangedEvents.length, 2);
extensionChangedEvent = extensionChangedEvents.last;
expect(extensionChangedEvent['extension'], 'ext.flutter.platformOverride');
expect(extensionChangedEvent['value'], 'macOS');
result = await hasReassemble(binding.testExtension(FoundationServiceExtensions.platformOverride.name, <String, String>{'value': 'android'}));
expect(result, <String, String>{'value': 'android'});
expect(binding.reassembled, 3);
expect(defaultTargetPlatform, TargetPlatform.android);
expect(extensionChangedEvents.length, 3);
extensionChangedEvent = extensionChangedEvents.last;
expect(extensionChangedEvent['extension'], 'ext.flutter.platformOverride');
expect(extensionChangedEvent['value'], 'android');
result = await hasReassemble(binding.testExtension(FoundationServiceExtensions.platformOverride.name, <String, String>{'value': 'fuchsia'}));
expect(result, <String, String>{'value': 'fuchsia'});
expect(binding.reassembled, 4);
expect(defaultTargetPlatform, TargetPlatform.fuchsia);
expect(extensionChangedEvents.length, 4);
extensionChangedEvent = extensionChangedEvents.last;
expect(extensionChangedEvent['extension'], 'ext.flutter.platformOverride');
expect(extensionChangedEvent['value'], 'fuchsia');
result = await hasReassemble(binding.testExtension(FoundationServiceExtensions.platformOverride.name, <String, String>{'value': 'default'}));
expect(result, <String, String>{'value': 'android'});
expect(binding.reassembled, 5);
expect(defaultTargetPlatform, TargetPlatform.android);
expect(extensionChangedEvents.length, 5);
extensionChangedEvent = extensionChangedEvents.last;
expect(extensionChangedEvent['extension'], 'ext.flutter.platformOverride');
expect(extensionChangedEvent['value'], 'android');
result = await hasReassemble(binding.testExtension(FoundationServiceExtensions.platformOverride.name, <String, String>{'value': 'iOS'}));
expect(result, <String, String>{'value': 'iOS'});
expect(binding.reassembled, 6);
expect(defaultTargetPlatform, TargetPlatform.iOS);
expect(extensionChangedEvents.length, 6);
extensionChangedEvent = extensionChangedEvents.last;
expect(extensionChangedEvent['extension'], 'ext.flutter.platformOverride');
expect(extensionChangedEvent['value'], 'iOS');
result = await hasReassemble(binding.testExtension(FoundationServiceExtensions.platformOverride.name, <String, String>{'value': 'linux'}));
expect(result, <String, String>{'value': 'linux'});
expect(binding.reassembled, 7);
expect(defaultTargetPlatform, TargetPlatform.linux);
expect(extensionChangedEvents.length, 7);
extensionChangedEvent = extensionChangedEvents.last;
expect(extensionChangedEvent['extension'], 'ext.flutter.platformOverride');
expect(extensionChangedEvent['value'], 'linux');
result = await hasReassemble(binding.testExtension(FoundationServiceExtensions.platformOverride.name, <String, String>{'value': 'windows'}));
expect(result, <String, String>{'value': 'windows'});
expect(binding.reassembled, 8);
expect(defaultTargetPlatform, TargetPlatform.windows);
expect(extensionChangedEvents.length, 8);
extensionChangedEvent = extensionChangedEvents.last;
expect(extensionChangedEvent['extension'], 'ext.flutter.platformOverride');
expect(extensionChangedEvent['value'], 'windows');
result = await hasReassemble(binding.testExtension(FoundationServiceExtensions.platformOverride.name, <String, String>{'value': 'bogus'}));
expect(result, <String, String>{'value': 'android'});
expect(binding.reassembled, 9);
expect(defaultTargetPlatform, TargetPlatform.android);
expect(extensionChangedEvents.length, 9);
extensionChangedEvent = extensionChangedEvents.last;
expect(extensionChangedEvent['extension'], 'ext.flutter.platformOverride');
expect(extensionChangedEvent['value'], 'android');
binding.reassembled = 0;
testedExtensions.add(FoundationServiceExtensions.platformOverride.name);
});
test('Service extensions - repaintRainbow', () async {
Map<String, dynamic> result;
Future<Map<String, dynamic>> pendingResult;
bool completed;
expect(binding.frameScheduled, isFalse);
expect(debugRepaintRainbowEnabled, false);
result = await binding.testExtension(RenderingServiceExtensions.repaintRainbow.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugRepaintRainbowEnabled, false);
expect(binding.frameScheduled, isFalse);
pendingResult = binding.testExtension(RenderingServiceExtensions.repaintRainbow.name, <String, String>{'enabled': 'true'});
completed = false;
pendingResult.whenComplete(() { completed = true; });
await binding.flushMicrotasks();
expect(completed, true);
expect(binding.frameScheduled, isFalse);
result = await pendingResult;
expect(result, <String, String>{'enabled': 'true'});
expect(debugRepaintRainbowEnabled, true);
result = await binding.testExtension(RenderingServiceExtensions.repaintRainbow.name, <String, String>{});
expect(result, <String, String>{'enabled': 'true'});
expect(debugRepaintRainbowEnabled, true);
expect(binding.frameScheduled, isFalse);
pendingResult = binding.testExtension(RenderingServiceExtensions.repaintRainbow.name, <String, String>{'enabled': 'false'});
completed = false;
pendingResult.whenComplete(() { completed = true; });
await binding.flushMicrotasks();
expect(completed, false);
expect(binding.frameScheduled, isTrue);
await binding.doFrame();
await binding.flushMicrotasks();
expect(completed, true);
expect(binding.frameScheduled, isFalse);
result = await pendingResult;
expect(result, <String, String>{'enabled': 'false'});
expect(debugRepaintRainbowEnabled, false);
result = await binding.testExtension(RenderingServiceExtensions.repaintRainbow.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugRepaintRainbowEnabled, false);
expect(binding.frameScheduled, isFalse);
testedExtensions.add(RenderingServiceExtensions.repaintRainbow.name);
});
test('Service extensions - debugDisableClipLayers', () async {
Map<String, dynamic> result;
Future<Map<String, dynamic>> pendingResult;
bool completed;
expect(binding.frameScheduled, isFalse);
expect(debugDisableClipLayers, false);
result = await binding.testExtension(RenderingServiceExtensions.debugDisableClipLayers.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugDisableClipLayers, false);
expect(binding.frameScheduled, isFalse);
pendingResult = binding.testExtension(RenderingServiceExtensions.debugDisableClipLayers.name, <String, String>{'enabled': 'true'});
completed = false;
pendingResult.whenComplete(() { completed = true; });
await binding.flushMicrotasks();
expect(binding.frameScheduled, isTrue);
expect(completed, isFalse);
await binding.doFrame();
await binding.flushMicrotasks();
expect(completed, isTrue);
expect(binding.frameScheduled, isFalse);
result = await pendingResult;
expect(result, <String, String>{'enabled': 'true'});
expect(debugDisableClipLayers, true);
result = await binding.testExtension(RenderingServiceExtensions.debugDisableClipLayers.name, <String, String>{});
expect(result, <String, String>{'enabled': 'true'});
expect(debugDisableClipLayers, true);
expect(binding.frameScheduled, isFalse);
pendingResult = binding.testExtension(RenderingServiceExtensions.debugDisableClipLayers.name, <String, String>{'enabled': 'false'});
await binding.flushMicrotasks();
expect(binding.frameScheduled, isTrue);
await binding.doFrame();
expect(binding.frameScheduled, isFalse);
result = await pendingResult;
expect(result, <String, String>{'enabled': 'false'});
expect(debugDisableClipLayers, false);
result = await binding.testExtension(RenderingServiceExtensions.debugDisableClipLayers.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugDisableClipLayers, false);
expect(binding.frameScheduled, isFalse);
testedExtensions.add(RenderingServiceExtensions.debugDisableClipLayers.name);
});
test('Service extensions - debugDisablePhysicalShapeLayers', () async {
Map<String, dynamic> result;
Future<Map<String, dynamic>> pendingResult;
bool completed;
expect(binding.frameScheduled, isFalse);
expect(debugDisablePhysicalShapeLayers, false);
result = await binding.testExtension(RenderingServiceExtensions.debugDisablePhysicalShapeLayers.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugDisablePhysicalShapeLayers, false);
expect(binding.frameScheduled, isFalse);
pendingResult = binding.testExtension(RenderingServiceExtensions.debugDisablePhysicalShapeLayers.name, <String, String>{'enabled': 'true'});
completed = false;
pendingResult.whenComplete(() { completed = true; });
await binding.flushMicrotasks();
expect(binding.frameScheduled, isTrue);
expect(completed, isFalse);
await binding.doFrame();
await binding.flushMicrotasks();
expect(completed, isTrue);
expect(binding.frameScheduled, isFalse);
result = await pendingResult;
expect(result, <String, String>{'enabled': 'true'});
expect(debugDisablePhysicalShapeLayers, true);
result = await binding.testExtension(RenderingServiceExtensions.debugDisablePhysicalShapeLayers.name, <String, String>{});
expect(result, <String, String>{'enabled': 'true'});
expect(debugDisablePhysicalShapeLayers, true);
expect(binding.frameScheduled, isFalse);
pendingResult = binding.testExtension(RenderingServiceExtensions.debugDisablePhysicalShapeLayers.name, <String, String>{'enabled': 'false'});
await binding.flushMicrotasks();
expect(binding.frameScheduled, isTrue);
await binding.doFrame();
expect(binding.frameScheduled, isFalse);
result = await pendingResult;
expect(result, <String, String>{'enabled': 'false'});
expect(debugDisablePhysicalShapeLayers, false);
result = await binding.testExtension(RenderingServiceExtensions.debugDisablePhysicalShapeLayers.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugDisablePhysicalShapeLayers, false);
expect(binding.frameScheduled, isFalse);
testedExtensions.add(RenderingServiceExtensions.debugDisablePhysicalShapeLayers.name);
});
test('Service extensions - debugDisableOpacityLayers', () async {
Map<String, dynamic> result;
Future<Map<String, dynamic>> pendingResult;
bool completed;
expect(binding.frameScheduled, isFalse);
expect(debugDisableOpacityLayers, false);
result = await binding.testExtension(RenderingServiceExtensions.debugDisableOpacityLayers.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugDisableOpacityLayers, false);
expect(binding.frameScheduled, isFalse);
pendingResult = binding.testExtension(RenderingServiceExtensions.debugDisableOpacityLayers.name, <String, String>{'enabled': 'true'});
completed = false;
pendingResult.whenComplete(() { completed = true; });
await binding.flushMicrotasks();
expect(binding.frameScheduled, isTrue);
expect(completed, isFalse);
await binding.doFrame();
await binding.flushMicrotasks();
expect(completed, isTrue);
expect(binding.frameScheduled, isFalse);
result = await pendingResult;
expect(result, <String, String>{'enabled': 'true'});
expect(debugDisableOpacityLayers, true);
result = await binding.testExtension(RenderingServiceExtensions.debugDisableOpacityLayers.name, <String, String>{});
expect(result, <String, String>{'enabled': 'true'});
expect(debugDisableOpacityLayers, true);
expect(binding.frameScheduled, isFalse);
pendingResult = binding.testExtension(RenderingServiceExtensions.debugDisableOpacityLayers.name, <String, String>{'enabled': 'false'});
await binding.flushMicrotasks();
expect(binding.frameScheduled, isTrue);
await binding.doFrame();
expect(binding.frameScheduled, isFalse);
result = await pendingResult;
expect(result, <String, String>{'enabled': 'false'});
expect(debugDisableOpacityLayers, false);
result = await binding.testExtension(RenderingServiceExtensions.debugDisableOpacityLayers.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(debugDisableOpacityLayers, false);
expect(binding.frameScheduled, isFalse);
testedExtensions.add(RenderingServiceExtensions.debugDisableOpacityLayers.name);
});
test('Service extensions - reassemble', () async {
Map<String, dynamic> result;
Future<Map<String, dynamic>> pendingResult;
bool completed;
completed = false;
expect(binding.reassembled, 0);
pendingResult = binding.testExtension(FoundationServiceExtensions.reassemble.name, <String, String>{});
pendingResult.whenComplete(() { completed = true; });
await binding.flushMicrotasks();
expect(binding.frameScheduled, isTrue);
expect(completed, false);
await binding.flushMicrotasks();
await binding.doFrame();
await binding.flushMicrotasks();
expect(completed, true);
expect(binding.frameScheduled, isFalse);
result = await pendingResult;
expect(result, <String, String>{});
expect(binding.reassembled, 1);
binding.reassembled = 0;
testedExtensions.add(FoundationServiceExtensions.reassemble.name);
});
test('Service extensions - showPerformanceOverlay', () async {
Map<String, dynamic> result;
// The performance overlay service extension is disabled on the web.
if (kIsWeb) {
expect(binding.extensions.containsKey(WidgetsServiceExtensions.showPerformanceOverlay.name), isFalse);
testedExtensions.add(WidgetsServiceExtensions.showPerformanceOverlay.name);
return;
}
expect(binding.frameScheduled, isFalse);
expect(WidgetsApp.showPerformanceOverlayOverride, false);
result = await binding.testExtension(WidgetsServiceExtensions.showPerformanceOverlay.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(WidgetsApp.showPerformanceOverlayOverride, false);
result = await binding.testExtension(WidgetsServiceExtensions.showPerformanceOverlay.name, <String, String>{'enabled': 'true'});
expect(result, <String, String>{'enabled': 'true'});
expect(WidgetsApp.showPerformanceOverlayOverride, true);
result = await binding.testExtension(WidgetsServiceExtensions.showPerformanceOverlay.name, <String, String>{});
expect(result, <String, String>{'enabled': 'true'});
expect(WidgetsApp.showPerformanceOverlayOverride, true);
result = await binding.testExtension(WidgetsServiceExtensions.showPerformanceOverlay.name, <String, String>{'enabled': 'false'});
expect(result, <String, String>{'enabled': 'false'});
expect(WidgetsApp.showPerformanceOverlayOverride, false);
result = await binding.testExtension(WidgetsServiceExtensions.showPerformanceOverlay.name, <String, String>{});
expect(result, <String, String>{'enabled': 'false'});
expect(WidgetsApp.showPerformanceOverlayOverride, false);
expect(binding.frameScheduled, isFalse);
testedExtensions.add(WidgetsServiceExtensions.showPerformanceOverlay.name);
});
test('Service extensions - timeDilation', () async {
final Iterable<Map<String, dynamic>> extensionChangedEvents = binding.getServiceExtensionStateChangedEvents('ext.flutter.timeDilation');
Map<String, dynamic> extensionChangedEvent;
Map<String, dynamic> result;
expect(binding.frameScheduled, isFalse);
expect(timeDilation, 1.0);
result = await binding.testExtension(SchedulerServiceExtensions.timeDilation.name, <String, String>{});
expect(result, <String, String>{SchedulerServiceExtensions.timeDilation.name: 1.0.toString()});
expect(timeDilation, 1.0);
expect(extensionChangedEvents, isEmpty);
result = await binding.testExtension(SchedulerServiceExtensions.timeDilation.name, <String, String>{SchedulerServiceExtensions.timeDilation.name: '100.0'});
expect(result, <String, String>{SchedulerServiceExtensions.timeDilation.name: 100.0.toString()});
expect(timeDilation, 100.0);
expect(extensionChangedEvents.length, 1);
extensionChangedEvent = extensionChangedEvents.last;
expect(extensionChangedEvent['extension'], 'ext.flutter.${SchedulerServiceExtensions.timeDilation.name}');
expect(extensionChangedEvent['value'], 100.0.toString());
result = await binding.testExtension(SchedulerServiceExtensions.timeDilation.name, <String, String>{});
expect(result, <String, String>{SchedulerServiceExtensions.timeDilation.name: 100.0.toString()});
expect(timeDilation, 100.0);
expect(extensionChangedEvents.length, 1);
result = await binding.testExtension(SchedulerServiceExtensions.timeDilation.name, <String, String>{SchedulerServiceExtensions.timeDilation.name: '1.0'});
expect(result, <String, String>{SchedulerServiceExtensions.timeDilation.name: 1.0.toString()});
expect(timeDilation, 1.0);
expect(extensionChangedEvents.length, 2);
extensionChangedEvent = extensionChangedEvents.last;
expect(extensionChangedEvent['extension'], 'ext.flutter.${SchedulerServiceExtensions.timeDilation.name}');
expect(extensionChangedEvent['value'], 1.0.toString());
result = await binding.testExtension(SchedulerServiceExtensions.timeDilation.name, <String, String>{});
expect(result, <String, String>{SchedulerServiceExtensions.timeDilation.name: 1.0.toString()});
expect(timeDilation, 1.0);
expect(extensionChangedEvents.length, 2);
expect(binding.frameScheduled, isFalse);
testedExtensions.add(SchedulerServiceExtensions.timeDilation.name);
});
test('Service extensions - brightnessOverride', () async {
Map<String, dynamic> result;
result = await binding.testExtension(FoundationServiceExtensions.brightnessOverride.name, <String, String>{});
final String brightnessValue = result['value'] as String;
expect(brightnessValue, 'Brightness.light');
testedExtensions.add(FoundationServiceExtensions.brightnessOverride.name);
});
test('Service extensions - activeDevToolsServerAddress', () async {
Map<String, dynamic> result;
result = await binding.testExtension(FoundationServiceExtensions.activeDevToolsServerAddress.name, <String, String>{});
String serverAddress = result['value'] as String;
expect(serverAddress, '');
result = await binding.testExtension(FoundationServiceExtensions.activeDevToolsServerAddress.name, <String, String>{'value': 'http://127.0.0.1:9101'});
serverAddress = result['value'] as String;
expect(serverAddress, 'http://127.0.0.1:9101');
result = await binding.testExtension(FoundationServiceExtensions.activeDevToolsServerAddress.name, <String, String>{'value': 'http://127.0.0.1:9102'});
serverAddress = result['value'] as String;
expect(serverAddress, 'http://127.0.0.1:9102');
testedExtensions.add(FoundationServiceExtensions.activeDevToolsServerAddress.name);
});
test('Service extensions - connectedVmServiceUri', () async {
Map<String, dynamic> result;
result = await binding.testExtension(FoundationServiceExtensions.connectedVmServiceUri.name, <String, String>{});
String serverAddress = result['value'] as String;
expect(serverAddress, '');
result = await binding.testExtension(FoundationServiceExtensions.connectedVmServiceUri.name, <String, String>{'value': 'http://127.0.0.1:54669/kMUMseKAnog=/'});
serverAddress = result['value'] as String;
expect(serverAddress, 'http://127.0.0.1:54669/kMUMseKAnog=/');
result = await binding.testExtension(FoundationServiceExtensions.connectedVmServiceUri.name, <String, String>{'value': 'http://127.0.0.1:54000/kMUMseKAnog=/'});
serverAddress = result['value'] as String;
expect(serverAddress, 'http://127.0.0.1:54000/kMUMseKAnog=/');
testedExtensions.add(FoundationServiceExtensions.connectedVmServiceUri.name);
});
}
| flutter/packages/flutter/test/foundation/service_extensions_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/foundation/service_extensions_test.dart",
"repo_id": "flutter",
"token_count": 16825
} | 684 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('wrapped HitTestResult gets HitTestEntry added to wrapping HitTestResult', () async {
final HitTestEntry entry1 = HitTestEntry(_DummyHitTestTarget());
final HitTestEntry entry2 = HitTestEntry(_DummyHitTestTarget());
final HitTestEntry entry3 = HitTestEntry(_DummyHitTestTarget());
final Matrix4 transform = Matrix4.translationValues(40.0, 150.0, 0.0);
final HitTestResult wrapped = MyHitTestResult()
..publicPushTransform(transform);
wrapped.add(entry1);
expect(wrapped.path, equals(<HitTestEntry>[entry1]));
expect(entry1.transform, transform);
final HitTestResult wrapping = HitTestResult.wrap(wrapped);
expect(wrapping.path, equals(<HitTestEntry>[entry1]));
expect(wrapping.path, same(wrapped.path));
wrapping.add(entry2);
expect(wrapping.path, equals(<HitTestEntry>[entry1, entry2]));
expect(wrapped.path, equals(<HitTestEntry>[entry1, entry2]));
expect(entry2.transform, transform);
wrapped.add(entry3);
expect(wrapping.path, equals(<HitTestEntry>[entry1, entry2, entry3]));
expect(wrapped.path, equals(<HitTestEntry>[entry1, entry2, entry3]));
expect(entry3.transform, transform);
});
test('HitTestResult should correctly push and pop transforms', () {
Matrix4? currentTransform(HitTestResult targetResult) {
final HitTestEntry entry = HitTestEntry(_DummyHitTestTarget());
targetResult.add(entry);
return entry.transform;
}
final MyHitTestResult result = MyHitTestResult();
final Matrix4 m1 = Matrix4.translationValues(10, 20, 0);
final Matrix4 m2 = Matrix4.rotationZ(1);
final Matrix4 m3 = Matrix4.diagonal3Values(1.1, 1.2, 1.0);
result.publicPushTransform(m1);
expect(currentTransform(result), equals(m1));
result.publicPushTransform(m2);
expect(currentTransform(result), equals(m2 * m1));
expect(currentTransform(result), equals(m2 * m1)); // Test repeated add
// The `wrapped` is wrapped at [m1, m2]
final MyHitTestResult wrapped = MyHitTestResult.wrap(result);
expect(currentTransform(wrapped), equals(m2 * m1));
result.publicPushTransform(m3);
// ignore: avoid_dynamic_calls
expect(currentTransform(result), equals(m3 * m2 * m1));
// ignore: avoid_dynamic_calls
expect(currentTransform(wrapped), equals(m3 * m2 * m1));
result.publicPopTransform();
result.publicPopTransform();
expect(currentTransform(result), equals(m1));
result.publicPopTransform();
result.publicPushTransform(m3);
expect(currentTransform(result), equals(m3));
result.publicPushTransform(m2);
expect(currentTransform(result), equals(m2 * m3));
});
test('HitTestResult should correctly push and pop offsets', () {
Matrix4? currentTransform(HitTestResult targetResult) {
final HitTestEntry entry = HitTestEntry(_DummyHitTestTarget());
targetResult.add(entry);
return entry.transform;
}
final MyHitTestResult result = MyHitTestResult();
final Matrix4 m1 = Matrix4.rotationZ(1);
final Matrix4 m2 = Matrix4.diagonal3Values(1.1, 1.2, 1.0);
const Offset o3 = Offset(10, 20);
final Matrix4 m3 = Matrix4.translationValues(o3.dx, o3.dy, 0.0);
// Test pushing offset as the first element
result.publicPushOffset(o3);
expect(currentTransform(result), equals(m3));
result.publicPopTransform();
result.publicPushOffset(o3);
result.publicPushTransform(m1);
expect(currentTransform(result), equals(m1 * m3));
expect(currentTransform(result), equals(m1 * m3)); // Test repeated add
// The `wrapped` is wrapped at [m1, m2]
final MyHitTestResult wrapped = MyHitTestResult.wrap(result);
expect(currentTransform(wrapped), equals(m1 * m3));
result.publicPushTransform(m2);
// ignore: avoid_dynamic_calls
expect(currentTransform(result), equals(m2 * m1 * m3));
// ignore: avoid_dynamic_calls
expect(currentTransform(wrapped), equals(m2 * m1 * m3));
result.publicPopTransform();
result.publicPopTransform();
result.publicPopTransform();
expect(currentTransform(result), equals(Matrix4.identity()));
result.publicPushTransform(m2);
result.publicPushOffset(o3);
result.publicPushTransform(m1);
// ignore: avoid_dynamic_calls
expect(currentTransform(result), equals(m1 * m3 * m2));
result.publicPopTransform();
expect(currentTransform(result), equals(m3 * m2));
});
}
class _DummyHitTestTarget implements HitTestTarget {
@override
void handleEvent(PointerEvent event, HitTestEntry entry) {
// Nothing to do.
}
}
class MyHitTestResult extends HitTestResult {
MyHitTestResult();
MyHitTestResult.wrap(super.result) : super.wrap();
void publicPushTransform(Matrix4 transform) => pushTransform(transform);
void publicPushOffset(Offset offset) => pushOffset(offset);
void publicPopTransform() => popTransform();
}
| flutter/packages/flutter/test/gestures/hit_test_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/gestures/hit_test_test.dart",
"repo_id": "flutter",
"token_count": 1747
} | 685 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('kTouchSlop is evaluated in the global coordinate space when scaled up', (WidgetTester tester) async {
int doubleTapCount = 0;
final Key redContainer = UniqueKey();
await tester.pumpWidget(
Center(
child: Transform.scale(
scale: 2.0,
child: GestureDetector(
onDoubleTap: () {
doubleTapCount++;
},
child: Container(
key: redContainer,
width: 100,
height: 150,
color: Colors.red,
),
),
),
),
);
// Move just below kTouchSlop should recognize tap.
final Offset center = tester.getCenter(find.byKey(redContainer));
TestGesture gesture = await tester.startGesture(center);
await gesture.up();
await tester.pump(kDoubleTapMinTime);
gesture = await tester.startGesture(center + const Offset(kDoubleTapSlop - 1, 0));
await gesture.up();
expect(doubleTapCount, 1);
doubleTapCount = 0;
gesture = await tester.startGesture(center);
await gesture.up();
await tester.pump(kDoubleTapMinTime);
gesture = await tester.startGesture(center + const Offset(kDoubleTapSlop + 1, 0));
await gesture.up();
expect(doubleTapCount, 0);
});
testWidgets('kTouchSlop is evaluated in the global coordinate space when scaled down', (WidgetTester tester) async {
int doubleTapCount = 0;
final Key redContainer = UniqueKey();
await tester.pumpWidget(
Center(
child: Transform.scale(
scale: 0.5,
child: GestureDetector(
onDoubleTap: () {
doubleTapCount++;
},
child: Container(
key: redContainer,
width: 500,
height: 500,
color: Colors.red,
),
),
),
),
);
// Move just below kTouchSlop should recognize tap.
final Offset center = tester.getCenter(find.byKey(redContainer));
TestGesture gesture = await tester.startGesture(center);
await gesture.up();
await tester.pump(kDoubleTapMinTime);
gesture = await tester.startGesture(center + const Offset(kDoubleTapSlop - 1, 0));
await gesture.up();
expect(doubleTapCount, 1);
doubleTapCount = 0;
gesture = await tester.startGesture(center);
await gesture.up();
await tester.pump(kDoubleTapMinTime);
gesture = await tester.startGesture(center + const Offset(kDoubleTapSlop + 1, 0));
await gesture.up();
expect(doubleTapCount, 0);
});
}
| flutter/packages/flutter/test/gestures/transformed_double_tap_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/gestures/transformed_double_tap_test.dart",
"repo_id": "flutter",
"token_count": 1299
} | 686 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/semantics_tester.dart';
import 'app_bar_utils.dart';
TextStyle? _iconStyle(WidgetTester tester, IconData icon) {
final RichText iconRichText = tester.widget<RichText>(
find.descendant(of: find.byIcon(icon).first, matching: find.byType(RichText)),
);
return iconRichText.text.style;
}
void main() {
setUp(() {
debugResetSemanticsIdCounter();
});
testWidgets('AppBar centers title on iOS', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(platform: TargetPlatform.android),
home: Scaffold(
appBar: AppBar(
title: const Text('X'),
),
),
),
);
final Finder title = find.text('X');
Offset center = tester.getCenter(title);
Size size = tester.getSize(title);
expect(center.dx, lessThan(400 - size.width / 2.0));
for (final TargetPlatform platform in <TargetPlatform>[TargetPlatform.iOS, TargetPlatform.macOS]) {
// Clear the widget tree to avoid animating between platforms.
await tester.pumpWidget(Container(key: UniqueKey()));
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(platform: platform),
home: Scaffold(
appBar: AppBar(
title: const Text('X'),
),
),
),
);
center = tester.getCenter(title);
size = tester.getSize(title);
expect(center.dx, greaterThan(400 - size.width / 2.0), reason: 'on ${platform.name}');
expect(center.dx, lessThan(400 + size.width / 2.0), reason: 'on ${platform.name}');
// One action is still centered.
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(platform: platform),
home: Scaffold(
appBar: AppBar(
title: const Text('X'),
actions: const <Widget>[
Icon(Icons.thumb_up),
],
),
),
),
);
center = tester.getCenter(title);
size = tester.getSize(title);
expect(center.dx, greaterThan(400 - size.width / 2.0), reason: 'on ${platform.name}');
expect(center.dx, lessThan(400 + size.width / 2.0), reason: 'on ${platform.name}');
// Two actions is left aligned again.
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(platform: platform),
home: Scaffold(
appBar: AppBar(
title: const Text('X'),
actions: const <Widget>[
Icon(Icons.thumb_up),
Icon(Icons.thumb_up),
],
),
),
),
);
center = tester.getCenter(title);
size = tester.getSize(title);
expect(center.dx, lessThan(400 - size.width / 2.0), reason: 'on ${platform.name}');
}
});
testWidgets('AppBar centerTitle:true centers on Android', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(platform: TargetPlatform.android),
home: Scaffold(
appBar: AppBar(
centerTitle: true,
title: const Text('X'),
),
),
),
);
final Finder title = find.text('X');
final Offset center = tester.getCenter(title);
final Size size = tester.getSize(title);
expect(center.dx, greaterThan(400 - size.width / 2.0));
expect(center.dx, lessThan(400 + size.width / 2.0));
});
testWidgets('AppBar centerTitle:false title start edge is 16.0 (LTR)', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
appBar: AppBar(
centerTitle: false,
title: const Placeholder(key: Key('X')),
),
),
),
);
final Finder titleWidget = find.byKey(const Key('X'));
expect(tester.getTopLeft(titleWidget).dx, 16.0);
expect(tester.getTopRight(titleWidget).dx, 800 - 16.0);
});
testWidgets('AppBar centerTitle:false title start edge is 16.0 (RTL)', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.rtl,
child: Scaffold(
appBar: AppBar(
centerTitle: false,
title: const Placeholder(key: Key('X')),
),
),
),
),
);
final Finder titleWidget = find.byKey(const Key('X'));
expect(tester.getTopRight(titleWidget).dx, 800.0 - 16.0);
expect(tester.getTopLeft(titleWidget).dx, 16.0);
});
testWidgets('AppBar titleSpacing:32 title start edge is 32.0 (LTR)', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
appBar: AppBar(
centerTitle: false,
titleSpacing: 32.0,
title: const Placeholder(key: Key('X')),
),
),
),
);
final Finder titleWidget = find.byKey(const Key('X'));
expect(tester.getTopLeft(titleWidget).dx, 32.0);
expect(tester.getTopRight(titleWidget).dx, 800 - 32.0);
});
testWidgets('AppBar titleSpacing:32 title start edge is 32.0 (RTL)', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.rtl,
child: Scaffold(
appBar: AppBar(
centerTitle: false,
titleSpacing: 32.0,
title: const Placeholder(key: Key('X')),
),
),
),
),
);
final Finder titleWidget = find.byKey(const Key('X'));
expect(tester.getTopRight(titleWidget).dx, 800.0 - 32.0);
expect(tester.getTopLeft(titleWidget).dx, 32.0);
});
testWidgets(
'AppBar centerTitle:false leading button title left edge is 72.0 (LTR)',
(WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
appBar: AppBar(
centerTitle: false,
title: const Text('X'),
),
// A drawer causes a leading hamburger.
drawer: const Drawer(),
),
),
);
expect(tester.getTopLeft(find.text('X')).dx, 72.0);
},
);
testWidgets(
'AppBar centerTitle:false leading button title left edge is 72.0 (RTL)',
(WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.rtl,
child: Scaffold(
appBar: AppBar(
centerTitle: false,
title: const Text('X'),
),
// A drawer causes a leading hamburger.
drawer: const Drawer(),
),
),
),
);
expect(tester.getTopRight(find.text('X')).dx, 800.0 - 72.0);
},
);
testWidgets('AppBar centerTitle:false title overflow OK', (WidgetTester tester) async {
// The app bar's title should be constrained to fit within the available space
// between the leading and actions widgets.
final Key titleKey = UniqueKey();
Widget leading = Container();
List<Widget> actions = <Widget>[];
Widget buildApp() {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
leading: leading,
centerTitle: false,
title: Container(
key: titleKey,
constraints: BoxConstraints.loose(const Size(1000.0, 1000.0)),
),
actions: actions,
),
),
);
}
await tester.pumpWidget(buildApp());
final Finder title = find.byKey(titleKey);
expect(tester.getTopLeft(title).dx, 72.0);
expect(
tester.getSize(title).width,
equals(
800.0 // Screen width.
- 56.0 // Leading button width.
- 16.0 // Leading button to title padding.
- 16.0, // Title right side padding.
),
);
actions = <Widget>[
const SizedBox(width: 100.0),
const SizedBox(width: 100.0),
];
await tester.pumpWidget(buildApp());
expect(tester.getTopLeft(title).dx, 72.0);
// The title shrinks by 200.0 to allow for the actions widgets.
expect(tester.getSize(title).width, equals(
800.0 // Screen width.
- 56.0 // Leading button width.
- 16.0 // Leading button to title padding.
- 16.0 // Title to actions padding
- 200.0,
)); // Actions' width.
leading = Container(); // AppBar will constrain the width to 24.0
await tester.pumpWidget(buildApp());
expect(tester.getTopLeft(title).dx, 72.0);
// Adding a leading widget shouldn't effect the title's size
expect(tester.getSize(title).width, equals(800.0 - 56.0 - 16.0 - 16.0 - 200.0));
});
testWidgets('AppBar centerTitle:true title overflow OK (LTR)', (WidgetTester tester) async {
// The app bar's title should be constrained to fit within the available space
// between the leading and actions widgets. When it's also centered it may
// also be start or end justified if it doesn't fit in the overall center.
final Key titleKey = UniqueKey();
double titleWidth = 700.0;
Widget? leading = Container();
List<Widget> actions = <Widget>[];
Widget buildApp() {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
leading: leading,
centerTitle: true,
title: Container(
key: titleKey,
constraints: BoxConstraints.loose(Size(titleWidth, 1000.0)),
),
actions: actions,
),
),
);
}
// Centering a title with width 700 within the 800 pixel wide test widget
// would mean that its start edge would have to be 50. The material spec says
// that the start edge of the title must be at least 72.
await tester.pumpWidget(buildApp());
final Finder title = find.byKey(titleKey);
expect(tester.getTopLeft(title).dx, 72.0);
expect(tester.getSize(title).width, equals(700.0));
// Centering a title with width 620 within the 800 pixel wide test widget
// would mean that its start edge would have to be 90. We reserve 72
// on the start and the padded actions occupy 96 on the end. That
// leaves 632, so the title is end justified but its width isn't changed.
await tester.pumpWidget(buildApp());
leading = null;
titleWidth = 620.0;
actions = <Widget>[
const SizedBox(width: 48.0),
const SizedBox(width: 48.0),
];
await tester.pumpWidget(buildApp());
expect(tester.getTopLeft(title).dx, 800 - 620 - 48 - 48 - 16);
expect(tester.getSize(title).width, equals(620.0));
});
testWidgets('AppBar centerTitle:true title overflow OK (RTL)', (WidgetTester tester) async {
// The app bar's title should be constrained to fit within the available space
// between the leading and actions widgets. When it's also centered it may
// also be start or end justified if it doesn't fit in the overall center.
final Key titleKey = UniqueKey();
double titleWidth = 700.0;
Widget? leading = Container();
List<Widget> actions = <Widget>[];
Widget buildApp() {
return MaterialApp(
home: Directionality(
textDirection: TextDirection.rtl,
child: Scaffold(
appBar: AppBar(
leading: leading,
centerTitle: true,
title: Container(
key: titleKey,
constraints: BoxConstraints.loose(Size(titleWidth, 1000.0)),
),
actions: actions,
),
),
),
);
}
// Centering a title with width 700 within the 800 pixel wide test widget
// would mean that its start edge would have to be 50. The material spec says
// that the start edge of the title must be at least 72.
await tester.pumpWidget(buildApp());
final Finder title = find.byKey(titleKey);
expect(tester.getTopRight(title).dx, 800.0 - 72.0);
expect(tester.getSize(title).width, equals(700.0));
// Centering a title with width 620 within the 800 pixel wide test widget
// would mean that its start edge would have to be 90. We reserve 72
// on the start and the padded actions occupy 96 on the end. That
// leaves 632, so the title is end justified but its width isn't changed.
await tester.pumpWidget(buildApp());
leading = null;
titleWidth = 620.0;
actions = <Widget>[
const SizedBox(width: 48.0),
const SizedBox(width: 48.0),
];
await tester.pumpWidget(buildApp());
expect(tester.getTopRight(title).dx, 620 + 48 + 48 + 16);
expect(tester.getSize(title).width, equals(620.0));
});
testWidgets('AppBar with no Scaffold', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: SizedBox(
height: kToolbarHeight,
child: AppBar(
leading: const Text('L'),
title: const Text('No Scaffold'),
actions: const <Widget>[Text('A1'), Text('A2')],
),
),
),
);
expect(find.text('L'), findsOneWidget);
expect(find.text('No Scaffold'), findsOneWidget);
expect(find.text('A1'), findsOneWidget);
expect(find.text('A2'), findsOneWidget);
});
testWidgets('AppBar render at zero size', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Center(
child: SizedBox.shrink(
child: Scaffold(
appBar: AppBar(
title: const Text('X'),
),
),
),
),
),
);
final Finder title = find.text('X');
expect(tester.getSize(title).isEmpty, isTrue);
});
testWidgets('AppBar actions are vertically centered', (WidgetTester tester) async {
final UniqueKey appBarKey = UniqueKey();
final UniqueKey leadingKey = UniqueKey();
final UniqueKey titleKey = UniqueKey();
final UniqueKey action0Key = UniqueKey();
final UniqueKey action1Key = UniqueKey();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
appBar: AppBar(
key: appBarKey,
leading: SizedBox(key: leadingKey, height: 50.0),
title: SizedBox(key: titleKey, height: 40.0),
actions: <Widget>[
SizedBox(key: action0Key, height: 20.0),
SizedBox(key: action1Key, height: 30.0),
],
),
),
),
);
// The vertical center of the widget with key, in global coordinates.
double yCenter(Key key) => tester.getCenter(find.byKey(key)).dy;
expect(yCenter(appBarKey), equals(yCenter(leadingKey)));
expect(yCenter(appBarKey), equals(yCenter(titleKey)));
expect(yCenter(appBarKey), equals(yCenter(action0Key)));
expect(yCenter(appBarKey), equals(yCenter(action1Key)));
});
testWidgets('AppBar drawer icon has default size', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('Howdy!'),
),
drawer: const Drawer(),
),
),
);
final double iconSize = const IconThemeData.fallback().size!;
expect(
tester.getSize(find.byIcon(Icons.menu)),
equals(Size(iconSize, iconSize)),
);
});
testWidgets('Material3 - AppBar drawer icon has default color', (WidgetTester tester) async {
final ThemeData themeData = ThemeData.from(
colorScheme: const ColorScheme.light(),
useMaterial3: true,
);
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
title: const Text('Howdy!'),
),
drawer: const Drawer(),
),
),
);
expect(_iconStyle(tester, Icons.menu)?.color, themeData.colorScheme.onSurfaceVariant);
});
testWidgets('AppBar drawer icon is sized by iconTheme', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('Howdy!'),
iconTheme: const IconThemeData(size: 30),
),
drawer: const Drawer(),
),
),
);
expect(
tester.getSize(find.byIcon(Icons.menu)),
equals(const Size(30, 30)),
);
});
testWidgets('AppBar drawer icon is colored by iconTheme', (WidgetTester tester) async {
final ThemeData themeData = ThemeData.from(colorScheme: const ColorScheme.light());
const Color color = Color(0xFF2196F3);
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
title: const Text('Howdy!'),
iconTheme: const IconThemeData(color: color),
),
drawer: const Drawer(),
),
),
);
expect(_iconStyle(tester, Icons.menu)?.color, color);
});
testWidgets('AppBar endDrawer icon has default size', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('Howdy!'),
),
endDrawer: const Drawer(),
),
),
);
final double iconSize = const IconThemeData.fallback().size!;
expect(
tester.getSize(find.byIcon(Icons.menu)),
equals(Size(iconSize, iconSize)),
);
});
testWidgets('Material3 - AppBar endDrawer icon has default color', (WidgetTester tester) async {
final ThemeData themeData = ThemeData.from(
colorScheme: const ColorScheme.light(),
useMaterial3: true,
);
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
title: const Text('Howdy!'),
),
endDrawer: const Drawer(),
),
),
);
expect(_iconStyle(tester, Icons.menu)?.color, themeData.colorScheme.onSurfaceVariant);
});
testWidgets('AppBar endDrawer icon is sized by iconTheme', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('Howdy!'),
iconTheme: const IconThemeData(size: 30),
),
endDrawer: const Drawer(),
),
),
);
expect(
tester.getSize(find.byIcon(Icons.menu)),
equals(const Size(30, 30)),
);
});
testWidgets('AppBar endDrawer icon is colored by iconTheme', (WidgetTester tester) async {
final ThemeData themeData = ThemeData.from(colorScheme: const ColorScheme.light());
const Color color = Color(0xFF2196F3);
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
title: const Text('Howdy!'),
iconTheme: const IconThemeData(color: color),
),
endDrawer: const Drawer(),
),
),
);
expect(_iconStyle(tester, Icons.menu)?.color, color);
});
testWidgets('Material3 - leading widget extends to edge and is square', (WidgetTester tester) async {
final ThemeData themeData = ThemeData(
platform: TargetPlatform.android,
useMaterial3: true,
);
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
leading: IconButton(icon: const Icon(Icons.menu), onPressed: () {}),
title: const Text('X'),
),
drawer: const Column(), // Doesn't really matter. Triggers a hamburger regardless.
),
),
);
// Default IconButton has a size of (48x48).
final Finder hamburger = find.byType(IconButton);
expect(tester.getTopLeft(hamburger), const Offset(4.0, 4.0));
expect(tester.getSize(hamburger), const Size(48.0, 48.0));
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
leading: Container(),
title: const Text('X'),
),
),
),
);
// Default leading widget has a size of (56x56).
final Finder leadingBox = find.byType(Container);
expect(tester.getTopLeft(leadingBox), Offset.zero);
expect(tester.getSize(leadingBox), const Size(56.0, 56.0));
// The custom leading widget should still be 56x56 even if its size is smaller.
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
leading: const SizedBox(height: 36, width: 36,),
title: const Text('X'),
), // Doesn't really matter. Triggers a hamburger regardless.
),
),
);
final Finder leading = find.byType(SizedBox);
expect(tester.getTopLeft(leading), Offset.zero);
expect(tester.getSize(leading), const Size(56.0, 56.0));
});
testWidgets('Material3 - Action is 4dp from edge and 48dp min', (WidgetTester tester) async {
final ThemeData theme = ThemeData(
platform: TargetPlatform.android,
useMaterial3: true,
);
await tester.pumpWidget(
MaterialApp(
theme: theme,
home: Scaffold(
appBar: AppBar(
title: const Text('X'),
actions: const <Widget> [
IconButton(
icon: Icon(Icons.share),
onPressed: null,
tooltip: 'Share',
iconSize: 20.0,
),
IconButton(
icon: Icon(Icons.add),
onPressed: null,
tooltip: 'Add',
iconSize: 60.0,
),
],
),
),
),
);
final Finder addButton = find.widgetWithIcon(IconButton, Icons.add);
expect(tester.getTopRight(addButton), const Offset(800.0, 0.0));
// It's still the size it was plus the 2 * 8dp padding from IconButton.
expect(tester.getSize(addButton), const Size(60.0 + 2 * 8.0, 56.0));
final Finder shareButton = find.widgetWithIcon(IconButton, Icons.share);
// The 20dp icon is expanded to fill the IconButton's touch target to 48dp.
expect(tester.getSize(shareButton), const Size(48.0, 48.0));
});
testWidgets('Material3 - AppBar uses the specified elevation or defaults to 0', (WidgetTester tester) async {
Widget buildAppBar([double? elevation]) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Scaffold(
appBar: AppBar(title: const Text('Title'), elevation: elevation),
),
);
}
Material getMaterial() => tester.widget<Material>(find.descendant(
of: find.byType(AppBar),
matching: find.byType(Material),
));
// Default elevation should be used for the material.
await tester.pumpWidget(buildAppBar());
expect(getMaterial().elevation, 0);
// AppBar should use the specified elevation.
await tester.pumpWidget(buildAppBar(8.0));
expect(getMaterial().elevation, 8.0);
});
testWidgets('scrolledUnderElevation', (WidgetTester tester) async {
Widget buildAppBar({double? elevation, double? scrolledUnderElevation}) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('Title'),
elevation: elevation,
scrolledUnderElevation: scrolledUnderElevation,
),
body: ListView.builder(
itemCount: 100,
itemBuilder: (BuildContext context, int index) => ListTile(title: Text('Item $index')),
),
),
);
}
Material getMaterial() => tester.widget<Material>(find.descendant(
of: find.byType(AppBar),
matching: find.byType(Material),
));
await tester.pumpWidget(buildAppBar(elevation: 2, scrolledUnderElevation: 10));
// Starts with the base elevation.
expect(getMaterial().elevation, 2);
await tester.fling(find.text('Item 2'), const Offset(0.0, -600.0), 2000.0);
await tester.pumpAndSettle();
// After scrolling it should be the scrolledUnderElevation.
expect(getMaterial().elevation, 10);
});
testWidgets('Material3 - scrolledUnderElevation with nested scroll view', (WidgetTester tester) async {
Widget buildAppBar({double? scrolledUnderElevation}) {
return MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Scaffold(
appBar: AppBar(
title: const Text('Title'),
scrolledUnderElevation: scrolledUnderElevation,
notificationPredicate: (ScrollNotification notification) {
return notification.depth == 1;
},
),
body: ListView.builder(
scrollDirection: Axis.horizontal,
itemCount: 4,
itemBuilder: (BuildContext context, int index) {
return SizedBox(
height: 600.0,
width: 800.0,
child: ListView.builder(
itemCount: 100,
itemBuilder: (BuildContext context, int index) =>
ListTile(title: Text('Item $index')),
),
);
},
),
),
);
}
Material getMaterial() => tester.widget<Material>(find.descendant(
of: find.byType(AppBar),
matching: find.byType(Material),
));
await tester.pumpWidget(buildAppBar(scrolledUnderElevation: 10));
// Starts with the base elevation.
expect(getMaterial().elevation, 0.0);
await tester.fling(find.text('Item 2'), const Offset(0.0, -600.0), 2000.0);
await tester.pumpAndSettle();
// After scrolling it should be the scrolledUnderElevation.
expect(getMaterial().elevation, 10);
});
testWidgets('AppBar dimensions, with and without bottom, primary', (WidgetTester tester) async {
const MediaQueryData topPadding100 = MediaQueryData(padding: EdgeInsets.only(top: 100.0));
await tester.pumpWidget(
Localizations(
locale: const Locale('en', 'US'),
delegates: const <LocalizationsDelegate<dynamic>>[
DefaultMaterialLocalizations.delegate,
DefaultWidgetsLocalizations.delegate,
],
child: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: topPadding100,
child: Scaffold(
primary: false,
appBar: AppBar(),
),
),
),
),
);
expect(appBarTop(tester), 0.0);
expect(appBarHeight(tester), kToolbarHeight);
await tester.pumpWidget(
Localizations(
locale: const Locale('en', 'US'),
delegates: const <LocalizationsDelegate<dynamic>>[
DefaultMaterialLocalizations.delegate,
DefaultWidgetsLocalizations.delegate,
],
child: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: topPadding100,
child: Scaffold(
appBar: AppBar(
title: const Text('title'),
),
),
),
),
),
);
expect(appBarTop(tester), 0.0);
expect(tester.getTopLeft(find.text('title')).dy, greaterThan(100.0));
expect(appBarHeight(tester), kToolbarHeight + 100.0);
await tester.pumpWidget(
Localizations(
locale: const Locale('en', 'US'),
delegates: const <LocalizationsDelegate<dynamic>>[
DefaultMaterialLocalizations.delegate,
DefaultWidgetsLocalizations.delegate,
],
child: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: topPadding100,
child: Scaffold(
primary: false,
appBar: AppBar(
bottom: PreferredSize(
preferredSize: const Size.fromHeight(200.0),
child: Container(),
),
),
),
),
),
),
);
expect(appBarTop(tester), 0.0);
expect(appBarHeight(tester), kToolbarHeight + 200.0);
await tester.pumpWidget(
Localizations(
locale: const Locale('en', 'US'),
delegates: const <LocalizationsDelegate<dynamic>>[
DefaultMaterialLocalizations.delegate,
DefaultWidgetsLocalizations.delegate,
],
child: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: topPadding100,
child: Scaffold(
appBar: AppBar(
bottom: PreferredSize(
preferredSize: const Size.fromHeight(200.0),
child: Container(),
),
),
),
),
),
),
);
expect(appBarTop(tester), 0.0);
expect(appBarHeight(tester), kToolbarHeight + 100.0 + 200.0);
await tester.pumpWidget(
Localizations(
locale: const Locale('en', 'US'),
delegates: const <LocalizationsDelegate<dynamic>>[
DefaultMaterialLocalizations.delegate,
DefaultWidgetsLocalizations.delegate,
],
child: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: topPadding100,
child: AppBar(
primary: false,
title: const Text('title'),
),
),
),
),
);
expect(appBarTop(tester), 0.0);
expect(tester.getTopLeft(find.text('title')).dy, lessThan(100.0));
});
testWidgets('AppBar in body excludes bottom SafeArea padding', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/26163
await tester.pumpWidget(
Localizations(
locale: const Locale('en', 'US'),
delegates: const <LocalizationsDelegate<dynamic>>[
DefaultMaterialLocalizations.delegate,
DefaultWidgetsLocalizations.delegate,
],
child: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(padding: EdgeInsets.symmetric(vertical: 100.0)),
child: Scaffold(
body: Column(
children: <Widget>[
AppBar(
title: const Text('title'),
),
],
),
),
),
),
),
);
expect(appBarTop(tester), 0.0);
expect(appBarHeight(tester), kToolbarHeight + 100.0);
});
testWidgets('AppBar.title sees the correct padding from MediaQuery', (WidgetTester tester) async {
bool titleBuilt = false;
await tester.pumpWidget(
Localizations(
locale: const Locale('en', 'US'),
delegates: const <LocalizationsDelegate<dynamic>>[
DefaultMaterialLocalizations.delegate,
DefaultWidgetsLocalizations.delegate,
],
child: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(padding: EdgeInsets.fromLTRB(12, 34, 56, 78)),
child: Scaffold(
appBar: AppBar(
title: Builder(builder: (BuildContext context) {
titleBuilt = true;
final EdgeInsets padding = MediaQuery.paddingOf(context);
expect(padding, EdgeInsets.zero);
return const Text('heh');
}),
),
),
),
),
),
);
expect(titleBuilt, isTrue);
});
testWidgets('AppBar updates when you add a drawer', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
appBar: AppBar(),
),
),
);
expect(find.byIcon(Icons.menu), findsNothing);
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
drawer: const Drawer(),
appBar: AppBar(),
),
),
);
expect(find.byIcon(Icons.menu), findsOneWidget);
});
testWidgets('AppBar does not draw menu for drawer if automaticallyImplyLeading is false', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
drawer: const Drawer(),
appBar: AppBar(
automaticallyImplyLeading: false,
),
),
),
);
expect(find.byIcon(Icons.menu), findsNothing);
});
testWidgets('AppBar does not update the leading if a route is popped case 1', (WidgetTester tester) async {
final Page<void> page1 = MaterialPage<void>(
key: const ValueKey<String>('1'),
child: Scaffold(
key: const ValueKey<String>('1'),
appBar: AppBar(),
),
);
final Page<void> page2 = MaterialPage<void>(
key: const ValueKey<String>('2'),
child: Scaffold(
key: const ValueKey<String>('2'),
appBar: AppBar(),
),
);
List<Page<void>> pages = <Page<void>>[ page1 ];
await tester.pumpWidget(
MaterialApp(
home: Navigator(
pages: pages,
onPopPage: (Route<dynamic> route, dynamic result) => false,
),
),
);
expect(find.byType(BackButton), findsNothing);
// Update pages
pages = <Page<void>>[ page2 ];
await tester.pumpWidget(
MaterialApp(
home: Navigator(
pages: pages,
onPopPage: (Route<dynamic> route, dynamic result) => false,
),
),
);
expect(find.byType(BackButton), findsNothing);
});
testWidgets('AppBar does not update the leading if a route is popped case 2', (WidgetTester tester) async {
final Page<void> page1 = MaterialPage<void>(
key: const ValueKey<String>('1'),
child: Scaffold(
key: const ValueKey<String>('1'),
appBar: AppBar(),
),
);
final Page<void> page2 = MaterialPage<void>(
key: const ValueKey<String>('2'),
child: Scaffold(
key: const ValueKey<String>('2'),
appBar: AppBar(),
),
);
List<Page<void>> pages = <Page<void>>[ page1, page2 ];
await tester.pumpWidget(
MaterialApp(
home: Navigator(
pages: pages,
onPopPage: (Route<dynamic> route, dynamic result) => false,
),
),
);
// The page2 should have a back button
expect(
find.descendant(
of: find.byKey(const ValueKey<String>('2')),
matching: find.byType(BackButton),
),
findsOneWidget,
);
// Update pages
pages = <Page<void>>[ page1 ];
await tester.pumpWidget(
MaterialApp(
home: Navigator(
pages: pages,
onPopPage: (Route<dynamic> route, dynamic result) => false,
),
),
);
await tester.pump(const Duration(milliseconds: 10));
// The back button should persist during the pop animation.
expect(
find.descendant(
of: find.byKey(const ValueKey<String>('2')),
matching: find.byType(BackButton),
),
findsOneWidget,
);
});
testWidgets('Material3 - AppBar ink splash draw on the correct canvas', (WidgetTester tester) async {
// This is a regression test for https://github.com/flutter/flutter/issues/58665
final Key key = UniqueKey();
await tester.pumpWidget(
MaterialApp(
// Test was designed against InkSplash so need to make sure that is used.
theme: ThemeData(
useMaterial3: true,
splashFactory: InkSplash.splashFactory
),
home: Center(
child: AppBar(
title: const Text('Abc'),
actions: <Widget>[
IconButton(
key: key,
icon: const Icon(Icons.add_circle),
tooltip: 'First button',
onPressed: () {},
),
],
flexibleSpace: DecoratedBox(
decoration: BoxDecoration(
gradient: LinearGradient(
begin: Alignment.topCenter,
end: const Alignment(-0.04, 1.0),
colors: <Color>[Colors.blue.shade500, Colors.blue.shade800],
),
),
),
),
),
),
);
final RenderObject painter = tester.renderObject(
find.descendant(
of: find.descendant(
of: find.byType(AppBar),
matching: find.byType(Stack),
),
matching: find.byType(Material).last,
),
);
await tester.tap(find.byKey(key));
expect(painter, paints..save()..translate()..save()..translate()..circle(x: 20.0, y: 20.0));
});
testWidgets('AppBar handles loose children 0', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
await tester.pumpWidget(
MaterialApp(
home: Center(
child: AppBar(
leading: Placeholder(key: key),
title: const Text('Abc'),
actions: const <Widget>[
Placeholder(fallbackWidth: 10.0),
Placeholder(fallbackWidth: 10.0),
Placeholder(fallbackWidth: 10.0),
],
),
),
),
);
expect(tester.renderObject<RenderBox>(find.byKey(key)).localToGlobal(Offset.zero), Offset.zero);
expect(tester.renderObject<RenderBox>(find.byKey(key)).size, const Size(56.0, 56.0));
});
testWidgets('AppBar handles loose children 1', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
await tester.pumpWidget(
MaterialApp(
home: Center(
child: AppBar(
leading: Placeholder(key: key),
title: const Text('Abc'),
actions: const <Widget>[
Placeholder(fallbackWidth: 10.0),
Placeholder(fallbackWidth: 10.0),
Placeholder(fallbackWidth: 10.0),
],
flexibleSpace: DecoratedBox(
decoration: BoxDecoration(
gradient: LinearGradient(
begin: Alignment.topCenter,
end: const Alignment(-0.04, 1.0),
colors: <Color>[Colors.blue.shade500, Colors.blue.shade800],
),
),
),
),
),
),
);
expect(tester.renderObject<RenderBox>(find.byKey(key)).localToGlobal(Offset.zero), Offset.zero);
expect(tester.renderObject<RenderBox>(find.byKey(key)).size, const Size(56.0, 56.0));
});
testWidgets('AppBar handles loose children 2', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
await tester.pumpWidget(
MaterialApp(
home: Center(
child: AppBar(
leading: Placeholder(key: key),
title: const Text('Abc'),
actions: const <Widget>[
Placeholder(fallbackWidth: 10.0),
Placeholder(fallbackWidth: 10.0),
Placeholder(fallbackWidth: 10.0),
],
flexibleSpace: DecoratedBox(
decoration: BoxDecoration(
gradient: LinearGradient(
begin: Alignment.topCenter,
end: const Alignment(-0.04, 1.0),
colors: <Color>[Colors.blue.shade500, Colors.blue.shade800],
),
),
),
bottom: PreferredSize(
preferredSize: const Size(0.0, kToolbarHeight),
child: Container(
height: 50.0,
padding: const EdgeInsets.all(4.0),
child: const Placeholder(
color: Color(0xFFFFFFFF),
),
),
),
),
),
),
);
expect(tester.renderObject<RenderBox>(find.byKey(key)).localToGlobal(Offset.zero), Offset.zero);
expect(tester.renderObject<RenderBox>(find.byKey(key)).size, const Size(56.0, 56.0));
});
testWidgets('AppBar handles loose children 3', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
await tester.pumpWidget(
MaterialApp(
home: Center(
child: AppBar(
leading: Placeholder(key: key),
title: const Text('Abc'),
actions: const <Widget>[
Placeholder(fallbackWidth: 10.0),
Placeholder(fallbackWidth: 10.0),
Placeholder(fallbackWidth: 10.0),
],
bottom: PreferredSize(
preferredSize: const Size(0.0, kToolbarHeight),
child: Container(
height: 50.0,
padding: const EdgeInsets.all(4.0),
child: const Placeholder(
color: Color(0xFFFFFFFF),
),
),
),
),
),
),
);
expect(tester.renderObject<RenderBox>(find.byKey(key)).localToGlobal(Offset.zero), Offset.zero);
expect(tester.renderObject<RenderBox>(find.byKey(key)).size, const Size(56.0, 56.0));
});
testWidgets('AppBar positioning of leading and trailing widgets with top padding', (WidgetTester tester) async {
const MediaQueryData topPadding100 = MediaQueryData(padding: EdgeInsets.only(top: 100));
final Key leadingKey = UniqueKey();
final Key titleKey = UniqueKey();
final Key trailingKey = UniqueKey();
await tester.pumpWidget(
Localizations(
locale: const Locale('en', 'US'),
delegates: const <LocalizationsDelegate<dynamic>>[
DefaultMaterialLocalizations.delegate,
DefaultWidgetsLocalizations.delegate,
],
child: Directionality(
textDirection: TextDirection.rtl,
child: MediaQuery(
data: topPadding100,
child: Scaffold(
primary: false,
appBar: AppBar(
leading: Placeholder(key: leadingKey), // Forced to 56x56, see _kLeadingWidth in app_bar.dart.
title: Placeholder(key: titleKey, fallbackHeight: kToolbarHeight),
actions: <Widget>[ Placeholder(key: trailingKey, fallbackWidth: 10) ],
),
),
),
),
),
);
expect(tester.getTopLeft(find.byType(AppBar)), Offset.zero);
expect(tester.getTopLeft(find.byKey(leadingKey)), const Offset(800.0 - 56.0, 100));
expect(tester.getTopLeft(find.byKey(trailingKey)), const Offset(0.0, 100));
// Because the topPadding eliminates the vertical space for the
// NavigationToolbar within the AppBar, the toolbar is constrained
// with minHeight=maxHeight=0. The _AppBarTitle widget vertically centers
// the title, so its Y coordinate relative to the toolbar is -kToolbarHeight / 2
// (-28). The top of the toolbar is at (screen coordinates) y=100, so the
// top of the title is 100 + -28 = 72. The toolbar clips its contents
// so the title isn't actually visible.
expect(tester.getTopLeft(find.byKey(titleKey)), const Offset(10 + NavigationToolbar.kMiddleSpacing, 72));
});
testWidgets('AppBar excludes header semantics correctly', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(
MaterialApp(
home: Center(
child: AppBar(
leading: const Text('Leading'),
title: const ExcludeSemantics(child: Text('Title')),
excludeHeaderSemantics: true,
actions: const <Widget>[
Text('Action 1'),
],
),
),
),
);
expect(semantics, hasSemantics(
TestSemantics.root(
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.scopesRoute],
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
label: 'Leading',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: 'Action 1',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
),
],
),
ignoreRect: true,
ignoreTransform: true,
ignoreId: true,
));
semantics.dispose();
});
testWidgets('Material3 - AppBar draws a light system bar for a dark background', (WidgetTester tester) async {
final ThemeData darkTheme = ThemeData.dark(useMaterial3: true);
await tester.pumpWidget(MaterialApp(
theme: darkTheme,
home: Scaffold(
appBar: AppBar(
title: const Text('test'),
),
),
));
expect(darkTheme.colorScheme.brightness, Brightness.dark);
expect(SystemChrome.latestStyle, const SystemUiOverlayStyle(
statusBarColor: Colors.transparent,
statusBarBrightness: Brightness.dark,
statusBarIconBrightness: Brightness.light,
));
});
testWidgets('Material3 - AppBar draws a dark system bar for a light background', (WidgetTester tester) async {
final ThemeData lightTheme = ThemeData(useMaterial3: true);
await tester.pumpWidget(
MaterialApp(
theme: lightTheme,
home: Scaffold(
appBar: AppBar(
title: const Text('test'),
),
),
),
);
expect(lightTheme.colorScheme.brightness, Brightness.light);
expect(SystemChrome.latestStyle, const SystemUiOverlayStyle(
statusBarColor: Colors.transparent,
statusBarBrightness: Brightness.light,
statusBarIconBrightness: Brightness.dark,
));
});
testWidgets('Material3 - Default system bar brightness based on AppBar background color brightness.', (WidgetTester tester) async {
Widget buildAppBar(ThemeData theme) {
return MaterialApp(
theme: theme,
home: Scaffold(
appBar: AppBar(title: const Text('Title')),
),
);
}
// Using a light theme.
{
await tester.pumpWidget(buildAppBar(ThemeData(useMaterial3: true)));
final Material appBarMaterial = tester.widget<Material>(
find.descendant(
of: find.byType(AppBar),
matching: find.byType(Material),
),
);
final Brightness appBarBrightness = ThemeData.estimateBrightnessForColor(appBarMaterial.color!);
final Brightness onAppBarBrightness = appBarBrightness == Brightness.light
? Brightness.dark
: Brightness.light;
expect(SystemChrome.latestStyle, SystemUiOverlayStyle(
statusBarColor: Colors.transparent,
statusBarBrightness: appBarBrightness,
statusBarIconBrightness: onAppBarBrightness,
));
}
// Using a dark theme.
{
await tester.pumpWidget(buildAppBar(ThemeData.dark(useMaterial3: true)));
final Material appBarMaterial = tester.widget<Material>(
find.descendant(
of: find.byType(AppBar),
matching: find.byType(Material),
),
);
final Brightness appBarBrightness = ThemeData.estimateBrightnessForColor(appBarMaterial.color!);
final Brightness onAppBarBrightness = appBarBrightness == Brightness.light
? Brightness.dark
: Brightness.light;
expect(SystemChrome.latestStyle, SystemUiOverlayStyle(
statusBarColor: Colors.transparent,
statusBarBrightness: appBarBrightness,
statusBarIconBrightness: onAppBarBrightness,
));
}
});
testWidgets('Material3 - Default status bar color', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
key: GlobalKey(),
theme: ThemeData.light().copyWith(
useMaterial3: true,
appBarTheme: const AppBarTheme(),
),
home: Scaffold(
appBar: AppBar(
title: const Text('title'),
),
),
),
);
expect(SystemChrome.latestStyle!.statusBarColor, Colors.transparent);
});
testWidgets('AppBar systemOverlayStyle is use to style status bar and navigation bar', (WidgetTester tester) async {
final SystemUiOverlayStyle systemOverlayStyle = SystemUiOverlayStyle.light.copyWith(
statusBarColor: Colors.red,
systemNavigationBarColor: Colors.green,
);
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('test'),
systemOverlayStyle: systemOverlayStyle,
),
),
),
);
expect(SystemChrome.latestStyle!.statusBarColor, Colors.red);
expect(SystemChrome.latestStyle!.systemNavigationBarColor, Colors.green);
});
testWidgets('AppBar shape default', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: AppBar(
leading: const Text('L'),
title: const Text('No Scaffold'),
actions: const <Widget>[Text('A1'), Text('A2')],
),
),
);
final Finder appBarFinder = find.byType(AppBar);
AppBar getAppBarWidget(Finder finder) => tester.widget<AppBar>(finder);
expect(getAppBarWidget(appBarFinder).shape, null);
final Finder materialFinder = find.byType(Material);
Material getMaterialWidget(Finder finder) => tester.widget<Material>(finder);
expect(getMaterialWidget(materialFinder).shape, null);
});
testWidgets('AppBar with shape', (WidgetTester tester) async {
const RoundedRectangleBorder roundedRectangleBorder = RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(15.0)),
);
await tester.pumpWidget(
MaterialApp(
home: AppBar(
leading: const Text('L'),
title: const Text('No Scaffold'),
actions: const <Widget>[Text('A1'), Text('A2')],
shape: roundedRectangleBorder,
),
),
);
final Finder appBarFinder = find.byType(AppBar);
AppBar getAppBarWidget(Finder finder) => tester.widget<AppBar>(finder);
expect(getAppBarWidget(appBarFinder).shape, roundedRectangleBorder);
final Finder materialFinder = find.byType(Material);
Material getMaterialWidget(Finder finder) => tester.widget<Material>(finder);
expect(getMaterialWidget(materialFinder).shape, roundedRectangleBorder);
});
testWidgets('AppBars title has upper limit on text scaling, textScaleFactor = 1, 1.34, 2', (WidgetTester tester) async {
late double textScaleFactor;
Widget buildFrame() {
return MaterialApp(
// Test designed against 2014 font sizes.
theme: ThemeData(textTheme: Typography.englishLike2014),
home: Builder(
builder: (BuildContext context) {
return MediaQuery.withClampedTextScaling(
minScaleFactor: textScaleFactor,
maxScaleFactor: textScaleFactor,
child: Scaffold(
appBar: AppBar(
centerTitle: false,
title: const Text('Jumbo', style: TextStyle(fontSize: 18)),
),
),
);
},
),
);
}
final Finder appBarTitle = find.text('Jumbo');
textScaleFactor = 1;
await tester.pumpWidget(buildFrame());
expect(tester.getRect(appBarTitle).height, 18);
textScaleFactor = 1.34;
await tester.pumpWidget(buildFrame());
expect(tester.getRect(appBarTitle).height, 24);
textScaleFactor = 2;
await tester.pumpWidget(buildFrame());
expect(tester.getRect(appBarTitle).height, 24);
});
testWidgets('AppBars with jumbo titles, textScaleFactor = 3, 3.5, 4', (WidgetTester tester) async {
double textScaleFactor = 1.0;
TextDirection textDirection = TextDirection.ltr;
bool centerTitle = false;
Widget buildFrame() {
return MaterialApp(
// Test designed against 2014 font sizes.
theme: ThemeData(textTheme: Typography.englishLike2014),
home: Builder(
builder: (BuildContext context) {
return Directionality(
textDirection: textDirection,
child: Builder(
builder: (BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: centerTitle,
title: MediaQuery.withClampedTextScaling(
minScaleFactor: textScaleFactor,
maxScaleFactor: textScaleFactor,
child: const Text('Jumbo'),
),
),
);
},
),
);
},
),
);
}
final Finder appBarTitle = find.text('Jumbo');
final Finder toolbar = find.byType(NavigationToolbar);
// Overall screen size is 800x600
// Left or right justified title is padded by 16 on the "start" side.
// Toolbar height is 56.
// "Jumbo" title is 100x20.
await tester.pumpWidget(buildFrame());
expect(tester.getRect(appBarTitle), const Rect.fromLTRB(16, 18, 116, 38));
expect(tester.getCenter(appBarTitle).dy, tester.getCenter(toolbar).dy);
textScaleFactor = 3; // "Jumbo" title is 300x60.
await tester.pumpWidget(buildFrame());
expect(tester.getRect(appBarTitle), const Rect.fromLTRB(16, -2, 316, 58));
expect(tester.getCenter(appBarTitle).dy, tester.getCenter(toolbar).dy);
textScaleFactor = 3.5; // "Jumbo" title is 350x70.
await tester.pumpWidget(buildFrame());
expect(tester.getRect(appBarTitle), const Rect.fromLTRB(16, -7, 366, 63));
expect(tester.getCenter(appBarTitle).dy, tester.getCenter(toolbar).dy);
textScaleFactor = 4; // "Jumbo" title is 400x80.
await tester.pumpWidget(buildFrame());
expect(tester.getRect(appBarTitle), const Rect.fromLTRB(16, -12, 416, 68));
expect(tester.getCenter(appBarTitle).dy, tester.getCenter(toolbar).dy);
textDirection = TextDirection.rtl; // Changed to rtl. "Jumbo" title is still 400x80.
await tester.pumpWidget(buildFrame());
expect(tester.getRect(appBarTitle), const Rect.fromLTRB(800.0 - 400.0 - 16.0, -12, 800.0 - 16.0, 68));
expect(tester.getCenter(appBarTitle).dy, tester.getCenter(toolbar).dy);
centerTitle = true; // Changed to true. "Jumbo" title is still 400x80.
await tester.pumpWidget(buildFrame());
expect(tester.getRect(appBarTitle), const Rect.fromLTRB(200, -12, 800.0 - 200.0, 68));
expect(tester.getCenter(appBarTitle).dy, tester.getCenter(toolbar).dy);
});
testWidgets('AppBar respects toolbarHeight', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('Title'),
toolbarHeight: 48,
),
body: Container(),
),
),
);
expect(appBarHeight(tester), 48);
});
testWidgets('AppBar respects leadingWidth', (WidgetTester tester) async {
const Key key = Key('leading');
await tester.pumpWidget(MaterialApp(
home: Scaffold(
appBar: AppBar(
leading: const Placeholder(key: key),
leadingWidth: 100,
title: const Text('Title'),
),
),
));
// By default toolbarHeight is 56.0.
expect(tester.getRect(find.byKey(key)), const Rect.fromLTRB(0, 0, 100, 56));
});
testWidgets("AppBar with EndDrawer doesn't have leading", (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Scaffold(
appBar: AppBar(),
endDrawer: const Drawer(),
),
));
final Finder endDrawerFinder = find.byTooltip('Open navigation menu');
await tester.tap(endDrawerFinder);
await tester.pump();
final Finder appBarFinder = find.byType(NavigationToolbar);
NavigationToolbar getAppBarWidget(Finder finder) => tester.widget<NavigationToolbar>(finder);
expect(getAppBarWidget(appBarFinder).leading, null);
});
testWidgets('AppBar.titleSpacing defaults to NavigationToolbar.kMiddleSpacing', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('Title'),
),
),
));
final NavigationToolbar navToolBar = tester.widget(find.byType(NavigationToolbar));
expect(navToolBar.middleSpacing, NavigationToolbar.kMiddleSpacing);
});
testWidgets('AppBar foregroundColor and backgroundColor', (WidgetTester tester) async {
const Color foregroundColor = Color(0xff00ff00);
const Color backgroundColor = Color(0xff00ffff);
final Key leadingIconKey = UniqueKey();
final Key actionIconKey = UniqueKey();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
appBar: AppBar(
foregroundColor: foregroundColor,
backgroundColor: backgroundColor,
leading: Icon(Icons.add_circle, key: leadingIconKey),
title: const Text('title'),
actions: <Widget>[Icon(Icons.ac_unit, key: actionIconKey), const Text('action')],
),
),
),
);
final Material appBarMaterial = tester.widget<Material>(
find.descendant(
of: find.byType(AppBar),
matching: find.byType(Material),
),
);
expect(appBarMaterial.color, backgroundColor);
final TextStyle titleTextStyle = tester.widget<DefaultTextStyle>(
find.ancestor(of: find.text('title'), matching: find.byType(DefaultTextStyle)).first,
).style;
expect(titleTextStyle.color, foregroundColor);
final IconThemeData leadingIconTheme = tester.widget<IconTheme>(
find.ancestor(of: find.byKey(leadingIconKey), matching: find.byType(IconTheme)).first,
).data;
expect(leadingIconTheme.color, foregroundColor);
final IconThemeData actionIconTheme = tester.widget<IconTheme>(
find.ancestor(of: find.byKey(actionIconKey), matching: find.byType(IconTheme)).first,
).data;
expect(actionIconTheme.color, foregroundColor);
// Test icon color
Color? leadingIconColor() => _iconStyle(tester, Icons.add_circle)?.color;
Color? actionIconColor() => _iconStyle(tester, Icons.ac_unit)?.color;
expect(leadingIconColor(), foregroundColor);
expect(actionIconColor(), foregroundColor);
});
testWidgets('Leading, title, and actions show correct default colors', (WidgetTester tester) async {
final ThemeData themeData = ThemeData.from(
colorScheme: const ColorScheme.light(
onPrimary: Colors.blue,
onSurface: Colors.red,
onSurfaceVariant: Colors.yellow),
);
final bool material3 = themeData.useMaterial3;
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
leading: const Icon(Icons.add_circle),
title: const Text('title'),
actions: const <Widget>[
Icon(Icons.ac_unit)
],
),
),
),
);
Color textColor() {
return tester.renderObject<RenderParagraph>(find.text('title')).text.style!.color!;
}
Color? leadingIconColor() => _iconStyle(tester, Icons.add_circle)?.color;
Color? actionIconColor() => _iconStyle(tester, Icons.ac_unit)?.color;
// M2 default color are onPrimary, and M3 has onSurface for leading and title,
// onSurfaceVariant for actions.
expect(textColor(), material3 ? Colors.red : Colors.blue);
expect(leadingIconColor(), material3 ? Colors.red : Colors.blue);
expect(actionIconColor(), material3 ? Colors.yellow : Colors.blue);
});
// Regression test for https://github.com/flutter/flutter/issues/107305
group('Material3 - Icons are colored correctly by IconTheme and ActionIconTheme', () {
testWidgets('Material3 - Icons and IconButtons are colored by IconTheme', (WidgetTester tester) async {
const Color iconColor = Color(0xff00ff00);
final Key leadingIconKey = UniqueKey();
final Key actionIconKey = UniqueKey();
await tester.pumpWidget(
MaterialApp(
theme: ThemeData.from(
colorScheme: const ColorScheme.light(), useMaterial3: true),
home: Scaffold(
appBar: AppBar(
iconTheme: const IconThemeData(color: iconColor),
leading: Icon(Icons.add_circle, key: leadingIconKey),
title: const Text('title'),
actions: <Widget>[
Icon(Icons.ac_unit, key: actionIconKey),
IconButton(icon: const Icon(Icons.add), onPressed: () {},)
],
),
),
),
);
Color? leadingIconColor() => _iconStyle(tester, Icons.add_circle)?.color;
Color? actionIconColor() => _iconStyle(tester, Icons.ac_unit)?.color;
Color? actionIconButtonColor() => _iconStyle(tester, Icons.add)?.color;
expect(leadingIconColor(), iconColor);
expect(actionIconColor(), iconColor);
expect(actionIconButtonColor(), iconColor);
});
testWidgets('Material3 - Action icons and IconButtons are colored by ActionIconTheme', (WidgetTester tester) async {
final ThemeData themeData = ThemeData.from(
colorScheme: const ColorScheme.light(),
useMaterial3: true,
);
const Color actionsIconColor = Color(0xff0000ff);
final Key leadingIconKey = UniqueKey();
final Key actionIconKey = UniqueKey();
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
actionsIconTheme: const IconThemeData(color: actionsIconColor),
leading: Icon(Icons.add_circle, key: leadingIconKey),
title: const Text('title'),
actions: <Widget>[
Icon(Icons.ac_unit, key: actionIconKey),
IconButton(icon: const Icon(Icons.add), onPressed: () {}),
],
),
),
),
);
Color? leadingIconColor() => _iconStyle(tester, Icons.add_circle)?.color;
Color? actionIconColor() => _iconStyle(tester, Icons.ac_unit)?.color;
Color? actionIconButtonColor() => _iconStyle(tester, Icons.add)?.color;
expect(leadingIconColor(), themeData.colorScheme.onSurface);
expect(actionIconColor(), actionsIconColor);
expect(actionIconButtonColor(), actionsIconColor);
});
testWidgets('Material3 - The actionIconTheme property overrides iconTheme', (WidgetTester tester) async {
final ThemeData themeData = ThemeData.from(
colorScheme: const ColorScheme.light(),
useMaterial3: true,
);
const Color overallIconColor = Color(0xff00ff00);
const Color actionsIconColor = Color(0xff0000ff);
final Key leadingIconKey = UniqueKey();
final Key actionIconKey = UniqueKey();
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
iconTheme: const IconThemeData(color: overallIconColor),
actionsIconTheme: const IconThemeData(color: actionsIconColor),
leading: Icon(Icons.add_circle, key: leadingIconKey),
title: const Text('title'),
actions: <Widget>[
Icon(Icons.ac_unit, key: actionIconKey),
IconButton(icon: const Icon(Icons.add), onPressed: () {}),
],
),
),
),
);
Color? leadingIconColor() => _iconStyle(tester, Icons.add_circle)?.color;
Color? actionIconColor() => _iconStyle(tester, Icons.ac_unit)?.color;
Color? actionIconButtonColor() => _iconStyle(tester, Icons.add)?.color;
expect(leadingIconColor(), overallIconColor);
expect(actionIconColor(), actionsIconColor);
expect(actionIconButtonColor(), actionsIconColor);
});
testWidgets('Material3 - AppBar.iconTheme should override any IconButtonTheme present in the theme', (WidgetTester tester) async {
final ThemeData themeData = ThemeData(
iconButtonTheme: IconButtonThemeData(
style: IconButton.styleFrom(
foregroundColor: Colors.red,
iconSize: 32.0,
),
),
useMaterial3: true,
);
const IconThemeData overallIconTheme = IconThemeData(color: Colors.yellow, size: 30.0);
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
iconTheme: overallIconTheme,
leading: IconButton(icon: const Icon(Icons.menu), onPressed: () {}),
title: const Text('title'),
actions: <Widget>[
IconButton(icon: const Icon(Icons.add), onPressed: () {}),
],
),
),
),
);
Color? leadingIconButtonColor() => _iconStyle(tester, Icons.menu)?.color;
double? leadingIconButtonSize() => _iconStyle(tester, Icons.menu)?.fontSize;
Color? actionIconButtonColor() => _iconStyle(tester, Icons.add)?.color;
double? actionIconButtonSize() => _iconStyle(tester, Icons.menu)?.fontSize;
expect(leadingIconButtonColor(), Colors.yellow);
expect(leadingIconButtonSize(), 30.0);
expect(actionIconButtonColor(), Colors.yellow);
expect(actionIconButtonSize(), 30.0);
});
testWidgets('Material3 - AppBar.iconTheme should override any IconButtonTheme present in the theme for widgets containing an iconButton', (WidgetTester tester) async {
final ThemeData themeData = ThemeData(
iconButtonTheme: IconButtonThemeData(
style: IconButton.styleFrom(
foregroundColor: Colors.red,
iconSize: 32.0,
),
),
useMaterial3: true,
);
const IconThemeData overallIconTheme = IconThemeData(color: Colors.yellow, size: 30.0);
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
iconTheme: overallIconTheme,
leading: BackButton(onPressed: () {}),
title: const Text('title'),
),
),
),
);
Color? leadingIconButtonColor() => _iconStyle(tester, Icons.arrow_back)?.color;
double? leadingIconButtonSize() => _iconStyle(tester, Icons.arrow_back)?.fontSize;
expect(leadingIconButtonColor(), Colors.yellow);
expect(leadingIconButtonSize(), 30.0);
});
testWidgets('Material3 - AppBar.actionsIconTheme should override any IconButtonTheme present in the theme', (WidgetTester tester) async {
final ThemeData themeData = ThemeData(
iconButtonTheme: IconButtonThemeData(
style: IconButton.styleFrom(
foregroundColor: Colors.red,
iconSize: 32.0,
),
),
useMaterial3: true,
);
const IconThemeData actionsIconTheme = IconThemeData(color: Colors.yellow, size: 30.0);
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
actionsIconTheme: actionsIconTheme,
title: const Text('title'),
leading: IconButton(icon: const Icon(Icons.menu), onPressed: () {}),
actions: <Widget>[
IconButton(icon: const Icon(Icons.add), onPressed: () {}),
],
),
),
),
);
Color? leadingIconButtonColor() => _iconStyle(tester, Icons.menu)?.color;
double? leadingIconButtonSize() => _iconStyle(tester, Icons.menu)?.fontSize;
Color? actionIconButtonColor() => _iconStyle(tester, Icons.add)?.color;
double? actionIconButtonSize() => _iconStyle(tester, Icons.add)?.fontSize;
// The leading icon button uses the style in the IconButtonTheme because only actionsIconTheme is provided.
expect(leadingIconButtonColor(), Colors.red);
expect(leadingIconButtonSize(), 32.0);
expect(actionIconButtonColor(), Colors.yellow);
expect(actionIconButtonSize(), 30.0);
});
testWidgets('Material3 - AppBar.actionsIconTheme should override any IconButtonTheme present in the theme for widgets containing an iconButton', (WidgetTester tester) async {
final ThemeData themeData = ThemeData(
iconButtonTheme: IconButtonThemeData(
style: IconButton.styleFrom(
foregroundColor: Colors.red,
iconSize: 32.0,
),
),
useMaterial3: true,
);
const IconThemeData actionsIconTheme = IconThemeData(color: Colors.yellow, size: 30.0);
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
actionsIconTheme: actionsIconTheme,
title: const Text('title'),
actions: <Widget>[
BackButton(onPressed: () {}),
],
),
),
),
);
Color? actionIconButtonColor() => _iconStyle(tester, Icons.arrow_back)?.color;
double? actionIconButtonSize() => _iconStyle(tester, Icons.arrow_back)?.fontSize;
expect(actionIconButtonColor(), Colors.yellow);
expect(actionIconButtonSize(), 30.0);
});
testWidgets('Material3 - The foregroundColor property of the AppBar overrides any IconButtonTheme present in the theme', (WidgetTester tester) async {
final ThemeData themeData = ThemeData(
iconButtonTheme: IconButtonThemeData(
style: IconButton.styleFrom(
foregroundColor: Colors.red,
),
),
useMaterial3: true,
);
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
foregroundColor: Colors.purple,
title: const Text('title'),
leading: IconButton(icon: const Icon(Icons.menu), onPressed: () {}),
actions: <Widget>[
IconButton(icon: const Icon(Icons.add), onPressed: () {}),
],
),
),
),
);
Color? leadingIconButtonColor() => _iconStyle(tester, Icons.menu)?.color;
Color? actionIconButtonColor() => _iconStyle(tester, Icons.add)?.color;
expect(leadingIconButtonColor(), Colors.purple);
expect(actionIconButtonColor(), Colors.purple);
});
// This is a regression test for https://github.com/flutter/flutter/issues/130485.
testWidgets('Material3 - AppBar.iconTheme is correctly applied in dark mode', (WidgetTester tester) async {
final ThemeData themeData = ThemeData(
colorScheme: const ColorScheme.dark().copyWith(onSurfaceVariant: Colors.red),
useMaterial3: true,
);
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
iconTheme: const IconThemeData(color: Colors.white),
leading: IconButton(icon: const Icon(Icons.menu), onPressed: () {}),
actions: <Widget>[
IconButton(icon: const Icon(Icons.add), onPressed: () {}),
],
),
),
),
);
Color? leadingIconButtonColor() => _iconStyle(tester, Icons.menu)?.color;
Color? actionIconButtonColor() => _iconStyle(tester, Icons.add)?.color;
expect(leadingIconButtonColor(), Colors.white);
expect(actionIconButtonColor(), Colors.white);
});
// This is a regression test for https://github.com/flutter/flutter/issues/130485.
testWidgets('Material3 - AppBar.foregroundColor is correctly applied in dark mode', (WidgetTester tester) async {
final ThemeData themeData = ThemeData(
colorScheme: const ColorScheme.dark().copyWith(onSurfaceVariant: Colors.red),
useMaterial3: true,
);
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
foregroundColor: Colors.white,
leading: IconButton(icon: const Icon(Icons.menu), onPressed: () {}),
actions: <Widget>[
IconButton(icon: const Icon(Icons.add), onPressed: () {}),
],
),
),
),
);
Color? leadingIconButtonColor() => _iconStyle(tester, Icons.menu)?.color;
Color? actionIconButtonColor() => _iconStyle(tester, Icons.add)?.color;
expect(leadingIconButtonColor(), Colors.white);
expect(actionIconButtonColor(), Colors.white);
});
// This is a regression test for https://github.com/flutter/flutter/issues/130485.
testWidgets('Material3 - AppBar.iconTheme is correctly applied in light mode', (WidgetTester tester) async {
final ThemeData themeData = ThemeData(
colorScheme: const ColorScheme.light().copyWith(onSurfaceVariant: Colors.red),
useMaterial3: true,
);
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
iconTheme: const IconThemeData(color: Colors.black87),
leading: IconButton(icon: const Icon(Icons.menu), onPressed: () {}),
actions: <Widget>[
IconButton(icon: const Icon(Icons.add), onPressed: () {}),
],
),
),
),
);
Color? leadingIconButtonColor() => _iconStyle(tester, Icons.menu)?.color;
Color? actionIconButtonColor() => _iconStyle(tester, Icons.add)?.color;
expect(leadingIconButtonColor(), Colors.black87);
expect(actionIconButtonColor(), Colors.black87);
});
// This is a regression test for https://github.com/flutter/flutter/issues/130485.
testWidgets('Material3 - AppBar.foregroundColor is correctly applied in light mode', (WidgetTester tester) async {
final ThemeData themeData = ThemeData(
colorScheme: const ColorScheme.light().copyWith(onSurfaceVariant: Colors.red),
useMaterial3: true,
);
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
foregroundColor: Colors.black87,
leading: IconButton(icon: const Icon(Icons.menu), onPressed: () {}),
actions: <Widget>[
IconButton(icon: const Icon(Icons.add), onPressed: () {}),
],
),
),
),
);
Color? leadingIconButtonColor() => _iconStyle(tester, Icons.menu)?.color;
Color? actionIconButtonColor() => _iconStyle(tester, Icons.add)?.color;
expect(leadingIconButtonColor(), Colors.black87);
expect(actionIconButtonColor(), Colors.black87);
});
});
group('MaterialStateColor scrolledUnder', () {
const Color scrolledColor = Color(0xff00ff00);
const Color defaultColor = Color(0xff0000ff);
Widget buildAppBar({
required double contentHeight,
bool reverse = false,
bool includeFlexibleSpace = false
}) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
elevation: 0,
backgroundColor: MaterialStateColor.resolveWith((Set<MaterialState> states) {
return states.contains(MaterialState.scrolledUnder)
? scrolledColor
: defaultColor;
}),
title: const Text('AppBar'),
flexibleSpace: includeFlexibleSpace
? const FlexibleSpaceBar(title: Text('FlexibleSpace'))
: null,
),
body: ListView(
reverse: reverse,
children: <Widget>[
Container(height: contentHeight, color: Colors.teal),
],
),
),
);
}
testWidgets('backgroundColor for horizontal scrolling', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
appBar: AppBar(
elevation: 0,
backgroundColor: MaterialStateColor.resolveWith((Set<MaterialState> states) {
return states.contains(MaterialState.scrolledUnder)
? scrolledColor
: defaultColor;
}),
title: const Text('AppBar'),
notificationPredicate: (ScrollNotification notification) {
// Represents both scroll views below being treated as a
// single viewport.
return notification.depth <= 1;
},
),
body: SingleChildScrollView(
child: SingleChildScrollView(
scrollDirection: Axis.horizontal,
child: Container(
height: 1200,
width: 1200,
color: Colors.teal,
),
),
),
),
)
);
expect(getAppBarBackgroundColor(tester), defaultColor);
expect(tester.getSize(findAppBarMaterial()).height, kToolbarHeight);
TestGesture gesture = await tester.startGesture(const Offset(50.0, 400.0));
await gesture.moveBy(const Offset(0.0, -kToolbarHeight));
await tester.pump();
await gesture.moveBy(const Offset(0.0, -kToolbarHeight));
await gesture.up();
await tester.pumpAndSettle();
expect(getAppBarBackgroundColor(tester), scrolledColor);
expect(tester.getSize(findAppBarMaterial()).height, kToolbarHeight);
gesture = await tester.startGesture(const Offset(50.0, 300.0));
// Scroll horizontally
await gesture.moveBy(const Offset(-kToolbarHeight, 0.0));
await tester.pump();
await gesture.moveBy(const Offset(-kToolbarHeight, 0.0));
await gesture.up();
await tester.pumpAndSettle();
// The app bar is still scrolled under vertically, so it should not have
// changed back in response to horizontal scrolling.
expect(getAppBarBackgroundColor(tester), scrolledColor);
expect(tester.getSize(findAppBarMaterial()).height, kToolbarHeight);
});
testWidgets('backgroundColor', (WidgetTester tester) async {
await tester.pumpWidget(
buildAppBar(contentHeight: 1200.0)
);
expect(getAppBarBackgroundColor(tester), defaultColor);
expect(tester.getSize(findAppBarMaterial()).height, kToolbarHeight);
TestGesture gesture = await tester.startGesture(const Offset(50.0, 400.0));
await gesture.moveBy(const Offset(0.0, -kToolbarHeight));
await gesture.up();
await tester.pumpAndSettle();
expect(getAppBarBackgroundColor(tester), scrolledColor);
expect(tester.getSize(findAppBarMaterial()).height, kToolbarHeight);
gesture = await tester.startGesture(const Offset(50.0, 300.0));
await gesture.moveBy(const Offset(0.0, kToolbarHeight));
await gesture.up();
await tester.pumpAndSettle();
expect(getAppBarBackgroundColor(tester), defaultColor);
expect(tester.getSize(findAppBarMaterial()).height, kToolbarHeight);
});
testWidgets('backgroundColor with FlexibleSpace', (WidgetTester tester) async {
await tester.pumpWidget(
buildAppBar(contentHeight: 1200.0, includeFlexibleSpace: true)
);
expect(getAppBarBackgroundColor(tester), defaultColor);
expect(tester.getSize(findAppBarMaterial()).height, kToolbarHeight);
TestGesture gesture = await tester.startGesture(const Offset(50.0, 400.0));
await gesture.moveBy(const Offset(0.0, -kToolbarHeight));
await gesture.up();
await tester.pumpAndSettle();
expect(getAppBarBackgroundColor(tester), scrolledColor);
expect(tester.getSize(findAppBarMaterial()).height, kToolbarHeight);
gesture = await tester.startGesture(const Offset(50.0, 300.0));
await gesture.moveBy(const Offset(0.0, kToolbarHeight));
await gesture.up();
await tester.pumpAndSettle();
expect(getAppBarBackgroundColor(tester), defaultColor);
expect(tester.getSize(findAppBarMaterial()).height, kToolbarHeight);
});
testWidgets('backgroundColor - reverse', (WidgetTester tester) async {
await tester.pumpWidget(
buildAppBar(contentHeight: 1200.0, reverse: true)
);
await tester.pump();
// In this test case, the content always extends under the AppBar, so it
// should always be the scrolledColor.
expect(getAppBarBackgroundColor(tester), scrolledColor);
expect(tester.getSize(findAppBarMaterial()).height, kToolbarHeight);
TestGesture gesture = await tester.startGesture(const Offset(50.0, 400.0));
await gesture.moveBy(const Offset(0.0, kToolbarHeight));
await gesture.up();
await tester.pumpAndSettle();
expect(getAppBarBackgroundColor(tester), scrolledColor);
expect(tester.getSize(findAppBarMaterial()).height, kToolbarHeight);
gesture = await tester.startGesture(const Offset(50.0, 300.0));
await gesture.moveBy(const Offset(0.0, -kToolbarHeight));
await gesture.up();
await tester.pumpAndSettle();
expect(getAppBarBackgroundColor(tester), scrolledColor);
expect(tester.getSize(findAppBarMaterial()).height, kToolbarHeight);
});
testWidgets('backgroundColor with FlexibleSpace - reverse', (WidgetTester tester) async {
await tester.pumpWidget(
buildAppBar(
contentHeight: 1200.0,
reverse: true,
includeFlexibleSpace: true,
)
);
await tester.pump();
// In this test case, the content always extends under the AppBar, so it
// should always be the scrolledColor.
expect(getAppBarBackgroundColor(tester), scrolledColor);
expect(tester.getSize(findAppBarMaterial()).height, kToolbarHeight);
TestGesture gesture = await tester.startGesture(const Offset(50.0, 400.0));
await gesture.moveBy(const Offset(0.0, kToolbarHeight));
await gesture.up();
await tester.pumpAndSettle();
expect(getAppBarBackgroundColor(tester), scrolledColor);
expect(tester.getSize(findAppBarMaterial()).height, kToolbarHeight);
gesture = await tester.startGesture(const Offset(50.0, 300.0));
await gesture.moveBy(const Offset(0.0, -kToolbarHeight));
await gesture.up();
await tester.pumpAndSettle();
expect(getAppBarBackgroundColor(tester), scrolledColor);
expect(tester.getSize(findAppBarMaterial()).height, kToolbarHeight);
});
testWidgets('_handleScrollNotification safely calls setState()', (WidgetTester tester) async {
// Regression test for failures found in Google internal issue b/185192049.
final ScrollController controller = ScrollController(initialScrollOffset: 400);
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('AppBar'),
),
body: Scrollbar(
thumbVisibility: true,
controller: controller,
child: ListView(
controller: controller,
children: <Widget>[
Container(height: 1200.0, color: Colors.teal),
],
),
),
),
),
);
expect(tester.takeException(), isNull);
controller.dispose();
});
testWidgets('does not trigger on horizontal scroll', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
appBar: AppBar(
elevation: 0,
backgroundColor: MaterialStateColor.resolveWith((Set<MaterialState> states) {
return states.contains(MaterialState.scrolledUnder)
? scrolledColor
: defaultColor;
}),
title: const Text('AppBar'),
),
body: ListView(
scrollDirection: Axis.horizontal,
children: <Widget>[
Container(height: 600.0, width: 1200.0, color: Colors.teal),
],
),
),
),
);
expect(getAppBarBackgroundColor(tester), defaultColor);
TestGesture gesture = await tester.startGesture(const Offset(50.0, 400.0));
await gesture.moveBy(const Offset(-100.0, 0.0));
await gesture.up();
await tester.pumpAndSettle();
expect(getAppBarBackgroundColor(tester), defaultColor);
gesture = await tester.startGesture(const Offset(50.0, 400.0));
await gesture.moveBy(const Offset(100.0, 0.0));
await gesture.up();
await tester.pumpAndSettle();
expect(getAppBarBackgroundColor(tester), defaultColor);
});
testWidgets('backgroundColor - not triggered in reverse for short content', (WidgetTester tester) async {
await tester.pumpWidget(
buildAppBar(
contentHeight: 200.0,
reverse: true,
)
);
await tester.pump();
// In reverse, the content here is not long enough to scroll under the app
// bar.
expect(getAppBarBackgroundColor(tester), defaultColor);
expect(tester.getSize(findAppBarMaterial()).height, kToolbarHeight);
final TestGesture gesture = await tester.startGesture(const Offset(50.0, 400.0));
await gesture.moveBy(const Offset(0.0, kToolbarHeight));
await gesture.up();
await tester.pumpAndSettle();
expect(getAppBarBackgroundColor(tester), defaultColor);
expect(tester.getSize(findAppBarMaterial()).height, kToolbarHeight);
});
testWidgets('backgroundColor with FlexibleSpace - not triggered in reverse for short content', (WidgetTester tester) async {
await tester.pumpWidget(
buildAppBar(
contentHeight: 200.0,
reverse: true,
includeFlexibleSpace: true,
)
);
await tester.pump();
// In reverse, the content here is not long enough to scroll under the app
// bar.
expect(getAppBarBackgroundColor(tester), defaultColor);
expect(tester.getSize(findAppBarMaterial()).height, kToolbarHeight);
final TestGesture gesture = await tester.startGesture(const Offset(50.0, 400.0));
await gesture.moveBy(const Offset(0.0, kToolbarHeight));
await gesture.up();
await tester.pumpAndSettle();
expect(getAppBarBackgroundColor(tester), defaultColor);
expect(tester.getSize(findAppBarMaterial()).height, kToolbarHeight);
});
});
// Regression test for https://github.com/flutter/flutter/issues/80256
testWidgets('The second page should have a back button even it has a end drawer', (WidgetTester tester) async {
final Page<void> page1 = MaterialPage<void>(
key: const ValueKey<String>('1'),
child: Scaffold(
key: const ValueKey<String>('1'),
appBar: AppBar(),
endDrawer: const Drawer(),
)
);
final Page<void> page2 = MaterialPage<void>(
key: const ValueKey<String>('2'),
child: Scaffold(
key: const ValueKey<String>('2'),
appBar: AppBar(),
endDrawer: const Drawer(),
)
);
final List<Page<void>> pages = <Page<void>>[ page1, page2 ];
await tester.pumpWidget(
MaterialApp(
home: Navigator(
pages: pages,
onPopPage: (Route<Object?> route, Object? result) => false,
),
),
);
// The page2 should have a back button.
expect(
find.descendant(
of: find.byKey(const ValueKey<String>('2')),
matching: find.byType(BackButton),
),
findsOneWidget
);
});
testWidgets('Only local entries that imply app bar dismissal will introduce an back button', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
key: key,
appBar: AppBar(),
),
),
);
expect(find.byType(BackButton), findsNothing);
// Push one entry that doesn't imply app bar dismissal.
ModalRoute.of(key.currentContext!)!.addLocalHistoryEntry(
LocalHistoryEntry(onRemove: () {}, impliesAppBarDismissal: false),
);
await tester.pump();
expect(find.byType(BackButton), findsNothing);
// Push one entry that implies app bar dismissal.
ModalRoute.of(key.currentContext!)!.addLocalHistoryEntry(
LocalHistoryEntry(onRemove: () {}),
);
await tester.pump();
expect(find.byType(BackButton), findsOneWidget);
});
testWidgets('AppBar.preferredHeightFor', (WidgetTester tester) async {
late double preferredHeight;
late Size preferredSize;
Widget buildFrame({ double? themeToolbarHeight, double? appBarToolbarHeight }) {
final AppBar appBar = AppBar(
toolbarHeight: appBarToolbarHeight,
);
return MaterialApp(
theme: ThemeData.light().copyWith(
appBarTheme: AppBarTheme(
toolbarHeight: themeToolbarHeight,
),
),
home: Builder(
builder: (BuildContext context) {
preferredHeight = AppBar.preferredHeightFor(context, appBar.preferredSize);
preferredSize = appBar.preferredSize;
return Scaffold(
appBar: appBar,
body: const Placeholder(),
);
},
),
);
}
await tester.pumpWidget(buildFrame());
expect(tester.getSize(find.byType(AppBar)).height, kToolbarHeight);
expect(preferredHeight, kToolbarHeight);
expect(preferredSize.height, kToolbarHeight);
await tester.pumpWidget(buildFrame(themeToolbarHeight: 96));
await tester.pumpAndSettle(); // Animate MaterialApp theme change.
expect(tester.getSize(find.byType(AppBar)).height, 96);
expect(preferredHeight, 96);
// Special case: AppBarTheme.toolbarHeight specified,
// AppBar.theme.toolbarHeight is null.
expect(preferredSize.height, kToolbarHeight);
await tester.pumpWidget(buildFrame(appBarToolbarHeight: 64));
await tester.pumpAndSettle(); // Animate MaterialApp theme change.
expect(tester.getSize(find.byType(AppBar)).height, 64);
expect(preferredHeight, 64);
expect(preferredSize.height, 64);
await tester.pumpWidget(buildFrame(appBarToolbarHeight: 64, themeToolbarHeight: 96));
await tester.pumpAndSettle(); // Animate MaterialApp theme change.
expect(tester.getSize(find.byType(AppBar)).height, 64);
expect(preferredHeight, 64);
expect(preferredSize.height, 64);
});
testWidgets('AppBar title with actions should have the same position regardless of centerTitle', (WidgetTester tester) async {
final Key titleKey = UniqueKey();
bool centerTitle = false;
Widget buildApp() {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
centerTitle: centerTitle,
title: Container(
key: titleKey,
constraints: BoxConstraints.loose(const Size(1000.0, 1000.0)),
),
actions: const <Widget>[
SizedBox(width: 48.0),
],
),
),
);
}
await tester.pumpWidget(buildApp());
final Finder title = find.byKey(titleKey);
expect(tester.getTopLeft(title).dx, 16.0);
centerTitle = true;
await tester.pumpWidget(buildApp());
expect(tester.getTopLeft(title).dx, 16.0);
});
testWidgets('AppBar leading widget can take up arbitrary space', (WidgetTester tester) async {
final Key leadingKey = UniqueKey();
final Key titleKey = UniqueKey();
late double leadingWidth;
Widget buildApp() {
return MaterialApp(
home: LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
leadingWidth = constraints.maxWidth / 2;
return Scaffold(
appBar: AppBar(
leading: Container(
key: leadingKey,
width: leadingWidth,
),
leadingWidth: leadingWidth,
title: Text('Title', key: titleKey),
),
);
}
),
);
}
await tester.pumpWidget(buildApp());
expect(tester.getTopLeft(find.byKey(titleKey)).dx, leadingWidth + 16.0);
expect(tester.getSize(find.byKey(leadingKey)).width, leadingWidth);
});
group('AppBar.forceMaterialTransparency', () {
Material getAppBarMaterial(WidgetTester tester) {
return tester.widget<Material>(find
.descendant(of: find.byType(AppBar), matching: find.byType(Material))
.first);
}
// Generates a MaterialApp with an AppBar with a TextButton beneath it
// (via extendBodyBehindAppBar = true).
Widget buildWidget({
required bool forceMaterialTransparency,
required VoidCallback onPressed
}) {
return MaterialApp(
home: Scaffold(
extendBodyBehindAppBar: true,
appBar: AppBar(
forceMaterialTransparency: forceMaterialTransparency,
elevation: 3,
backgroundColor: Colors.red,
title: const Text('AppBar'),
),
body: Align(
alignment: Alignment.topCenter,
child: TextButton(
onPressed: onPressed,
child: const Text('press me'),
),
),
),
);
}
testWidgets(
'forceMaterialTransparency == true allows gestures beneath the app bar', (WidgetTester tester) async {
bool buttonWasPressed = false;
final Widget widget = buildWidget(
forceMaterialTransparency:true,
onPressed:() { buttonWasPressed = true; },
);
await tester.pumpWidget(widget);
final Material material = getAppBarMaterial(tester);
expect(material.type, MaterialType.transparency);
final Finder buttonFinder = find.byType(TextButton);
await tester.tap(buttonFinder);
await tester.pump();
expect(buttonWasPressed, isTrue);
});
testWidgets(
'forceMaterialTransparency == false does not allow gestures beneath the app bar',
(WidgetTester tester) async {
// Set this, and tester.tap(warnIfMissed:false), to suppress
// errors/warning that the button is not hittable (which is expected).
WidgetController.hitTestWarningShouldBeFatal = false;
bool buttonWasPressed = false;
final Widget widget = buildWidget(
forceMaterialTransparency:false,
onPressed:() { buttonWasPressed = true; },
);
await tester.pumpWidget(widget);
final Material material = getAppBarMaterial(tester);
expect(material.type, MaterialType.canvas);
final Finder buttonFinder = find.byType(TextButton);
await tester.tap(buttonFinder, warnIfMissed:false);
await tester.pump();
expect(buttonWasPressed, isFalse);
});
});
group('Material 2', () {
testWidgets('Material2 - AppBar draws a light system bar for a dark background', (WidgetTester tester) async {
final ThemeData darkTheme = ThemeData.dark(useMaterial3: false);
await tester.pumpWidget(MaterialApp(
theme: darkTheme,
home: Scaffold(
appBar: AppBar(
title: const Text('test'),
),
),
));
expect(darkTheme.colorScheme.brightness, Brightness.dark);
expect(SystemChrome.latestStyle, const SystemUiOverlayStyle(
statusBarBrightness: Brightness.dark,
statusBarIconBrightness: Brightness.light,
));
});
testWidgets('Material2 - AppBar drawer icon has default color', (WidgetTester tester) async {
final ThemeData themeData = ThemeData.from(
colorScheme: const ColorScheme.light(),
useMaterial3: false,
);
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
title: const Text('Howdy!'),
),
drawer: const Drawer(),
),
),
);
expect(_iconStyle(tester, Icons.menu)?.color, themeData.colorScheme.onPrimary);
});
testWidgets('Material2 - AppBar endDrawer icon has default color', (WidgetTester tester) async {
final ThemeData themeData = ThemeData.from(
colorScheme: const ColorScheme.light(),
useMaterial3: false,
);
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
title: const Text('Howdy!'),
),
endDrawer: const Drawer(),
),
),
);
expect(_iconStyle(tester, Icons.menu)?.color, themeData.colorScheme.onPrimary);
});
testWidgets('Material2 - leading widget extends to edge and is square', (WidgetTester tester) async {
final ThemeData themeData = ThemeData(
platform: TargetPlatform.android,
useMaterial3: false,
);
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
leading: IconButton(icon: const Icon(Icons.menu), onPressed: () {}),
title: const Text('X'),
),
drawer: const Column(), // Doesn't really matter. Triggers a hamburger regardless.
),
),
);
// Default IconButton has a size of (56x56).
final Finder hamburger = find.byType(IconButton);
expect(tester.getTopLeft(hamburger), Offset.zero);
expect(tester.getSize(hamburger), const Size(56.0, 56.0));
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
leading: Container(),
title: const Text('X'),
),
),
),
);
// Default leading widget has a size of (56x56).
final Finder leadingBox = find.byType(Container);
expect(tester.getTopLeft(leadingBox), Offset.zero);
expect(tester.getSize(leadingBox), const Size(56.0, 56.0));
// The custom leading widget should still be 56x56 even if its size is smaller.
await tester.pumpWidget(
MaterialApp(
theme: themeData,
home: Scaffold(
appBar: AppBar(
leading: const SizedBox(height: 36, width: 36,),
title: const Text('X'),
), // Doesn't really matter. Triggers a hamburger regardless.
),
),
);
final Finder leading = find.byType(SizedBox);
expect(tester.getTopLeft(leading), Offset.zero);
expect(tester.getSize(leading), const Size(56.0, 56.0));
});
testWidgets('Material2 - Action is 4dp from edge and 48dp min', (WidgetTester tester) async {
final ThemeData theme = ThemeData(
platform: TargetPlatform.android,
useMaterial3: false,
);
await tester.pumpWidget(
MaterialApp(
theme: theme,
home: Scaffold(
appBar: AppBar(
title: const Text('X'),
actions: const <Widget> [
IconButton(
icon: Icon(Icons.share),
onPressed: null,
tooltip: 'Share',
iconSize: 20.0,
),
IconButton(
icon: Icon(Icons.add),
onPressed: null,
tooltip: 'Add',
iconSize: 60.0,
),
],
),
),
),
);
final Finder addButton = find.widgetWithIcon(IconButton, Icons.add);
expect(tester.getTopRight(addButton), const Offset(800.0, 0.0));
// It's still the size it was plus the 2 * 8dp padding from IconButton.
expect(tester.getSize(addButton), const Size(60.0 + 2 * 8.0, 56.0));
final Finder shareButton = find.widgetWithIcon(IconButton, Icons.share);
// The 20dp icon is expanded to fill the IconButton's touch target to 48dp.
expect(tester.getSize(shareButton), const Size(48.0, 56.0));
});
testWidgets('Material2 - AppBar uses the specified elevation or defaults to 4.0', (WidgetTester tester) async {
Widget buildAppBar([double? elevation]) {
return MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Scaffold(
appBar: AppBar(title: const Text('Title'), elevation: elevation),
),
);
}
Material getMaterial() => tester.widget<Material>(find.descendant(
of: find.byType(AppBar),
matching: find.byType(Material),
));
// Default elevation should be used for the material.
await tester.pumpWidget(buildAppBar());
expect(getMaterial().elevation, 4);
// AppBar should use the specified elevation.
await tester.pumpWidget(buildAppBar(8.0));
expect(getMaterial().elevation, 8.0);
});
testWidgets('Material2 - AppBar ink splash draw on the correct canvas', (WidgetTester tester) async {
// This is a regression test for https://github.com/flutter/flutter/issues/58665
final Key key = UniqueKey();
await tester.pumpWidget(
MaterialApp(
// Test was designed against InkSplash so need to make sure that is used.
theme: ThemeData(
useMaterial3: false,
splashFactory: InkSplash.splashFactory
),
home: Center(
child: AppBar(
title: const Text('Abc'),
actions: <Widget>[
IconButton(
key: key,
icon: const Icon(Icons.add_circle),
tooltip: 'First button',
onPressed: () {},
),
],
flexibleSpace: DecoratedBox(
decoration: BoxDecoration(
gradient: LinearGradient(
begin: Alignment.topCenter,
end: const Alignment(-0.04, 1.0),
colors: <Color>[Colors.blue.shade500, Colors.blue.shade800],
),
),
),
),
),
),
);
final RenderObject painter = tester.renderObject(
find.descendant(
of: find.descendant(
of: find.byType(AppBar),
matching: find.byType(Stack),
),
matching: find.byType(Material),
),
);
await tester.tap(find.byKey(key));
expect(painter, paints..save()..translate()..save()..translate()..circle(x: 24.0, y: 28.0));
});
testWidgets('Material2 - Default status bar color', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
key: GlobalKey(),
theme: ThemeData.light().copyWith(
useMaterial3: false,
appBarTheme: const AppBarTheme(),
),
home: Scaffold(
appBar: AppBar(
title: const Text('title'),
),
),
),
);
expect(SystemChrome.latestStyle!.statusBarColor, null);
});
testWidgets('Material2 - AppBar draws a dark system bar for a light background', (WidgetTester tester) async {
final ThemeData lightTheme = ThemeData(primarySwatch: Colors.lightBlue, useMaterial3: false);
await tester.pumpWidget(
MaterialApp(
theme: lightTheme,
home: Scaffold(
appBar: AppBar(
title: const Text('test'),
),
),
),
);
expect(lightTheme.colorScheme.brightness, Brightness.light);
expect(SystemChrome.latestStyle, const SystemUiOverlayStyle(
statusBarBrightness: Brightness.light,
statusBarIconBrightness: Brightness.dark,
));
});
testWidgets('Material2 - Default system bar brightness based on AppBar background color brightness.', (WidgetTester tester) async {
Widget buildAppBar(ThemeData theme) {
return MaterialApp(
theme: theme,
home: Scaffold(
appBar: AppBar(title: const Text('Title')),
),
);
}
// Using a light theme.
{
await tester.pumpWidget(buildAppBar(ThemeData(useMaterial3: false)));
final Material appBarMaterial = tester.widget<Material>(
find.descendant(
of: find.byType(AppBar),
matching: find.byType(Material),
),
);
final Brightness appBarBrightness = ThemeData.estimateBrightnessForColor(appBarMaterial.color!);
final Brightness onAppBarBrightness = appBarBrightness == Brightness.light
? Brightness.dark
: Brightness.light;
expect(SystemChrome.latestStyle, SystemUiOverlayStyle(
statusBarBrightness: appBarBrightness,
statusBarIconBrightness: onAppBarBrightness,
));
}
// Using a dark theme.
{
await tester.pumpWidget(buildAppBar(ThemeData.dark(useMaterial3: false)));
final Material appBarMaterial = tester.widget<Material>(
find.descendant(
of: find.byType(AppBar),
matching: find.byType(Material),
),
);
final Brightness appBarBrightness = ThemeData.estimateBrightnessForColor(appBarMaterial.color!);
final Brightness onAppBarBrightness = appBarBrightness == Brightness.light
? Brightness.dark
: Brightness.light;
expect(SystemChrome.latestStyle, SystemUiOverlayStyle(
statusBarBrightness: appBarBrightness,
statusBarIconBrightness: onAppBarBrightness,
));
}
});
});
}
| flutter/packages/flutter/test/material/app_bar_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/app_bar_test.dart",
"repo_id": "flutter",
"token_count": 46711
} | 687 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/semantics_tester.dart';
void main() {
// Pumps and ensures that the BottomSheet animates non-linearly.
Future<void> checkNonLinearAnimation(WidgetTester tester) async {
final Offset firstPosition = tester.getCenter(find.text('BottomSheet'));
await tester.pump(const Duration(milliseconds: 30));
final Offset secondPosition = tester.getCenter(find.text('BottomSheet'));
await tester.pump(const Duration(milliseconds: 30));
final Offset thirdPosition = tester.getCenter(find.text('BottomSheet'));
final double dyDelta1 = secondPosition.dy - firstPosition.dy;
final double dyDelta2 = thirdPosition.dy - secondPosition.dy;
// If the animation were linear, these two values would be the same.
expect(dyDelta1, isNot(moreOrLessEquals(dyDelta2, epsilon: 0.1)));
}
testWidgets('Throw if enable drag without an animation controller', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/89168
await tester.pumpWidget(
MaterialApp(
home: BottomSheet(
onClosing: () {},
builder: (_) => Container(
height: 200,
color: Colors.red,
child: const Text('BottomSheet'),
),
),
),
);
final FlutterExceptionHandler? handler = FlutterError.onError;
FlutterErrorDetails? error;
FlutterError.onError = (FlutterErrorDetails details) {
error = details;
};
await tester.drag(find.text('BottomSheet'), const Offset(0.0, 150.0));
expect(error, isNotNull);
FlutterError.onError = handler;
});
testWidgets('Disposing app while bottom sheet is disappearing does not crash', (WidgetTester tester) async {
late BuildContext savedContext;
await tester.pumpWidget(
MaterialApp(
home: Builder(
builder: (BuildContext context) {
savedContext = context;
return Container();
},
),
),
);
await tester.pump();
expect(find.text('BottomSheet'), findsNothing);
// Bring up bottom sheet.
bool showBottomSheetThenCalled = false;
showModalBottomSheet<void>(
context: savedContext,
builder: (BuildContext context) => const Text('BottomSheet'),
).then<void>((void value) {
showBottomSheetThenCalled = true;
});
await tester.pumpAndSettle();
expect(find.text('BottomSheet'), findsOneWidget);
expect(showBottomSheetThenCalled, isFalse);
// Start closing animation of Bottom sheet.
tester.state<NavigatorState>(find.byType(Navigator)).pop();
await tester.pump();
// Dispose app by replacing it with a container. This shouldn't crash.
await tester.pumpWidget(Container());
});
testWidgets('Swiping down a BottomSheet should dismiss it by default', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
bool showBottomSheetThenCalled = false;
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
await tester.pump();
expect(showBottomSheetThenCalled, isFalse);
expect(find.text('BottomSheet'), findsNothing);
scaffoldKey.currentState!.showBottomSheet((BuildContext context) {
return const SizedBox(
height: 200.0,
child: Text('BottomSheet'),
);
}).closed.whenComplete(() {
showBottomSheetThenCalled = true;
});
await tester.pumpAndSettle();
expect(showBottomSheetThenCalled, isFalse);
expect(find.text('BottomSheet'), findsOneWidget);
// Swipe the bottom sheet to dismiss it.
await tester.drag(find.text('BottomSheet'), const Offset(0.0, 150.0));
await tester.pumpAndSettle(); // Bottom sheet dismiss animation.
expect(showBottomSheetThenCalled, isTrue);
expect(find.text('BottomSheet'), findsNothing);
});
testWidgets('Swiping down a BottomSheet should not dismiss it when enableDrag is false', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
bool showBottomSheetThenCalled = false;
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
await tester.pump();
expect(showBottomSheetThenCalled, isFalse);
expect(find.text('BottomSheet'), findsNothing);
scaffoldKey.currentState!.showBottomSheet((BuildContext context) {
return const SizedBox(
height: 200.0,
child: Text('BottomSheet'),
);
},
enableDrag: false
).closed.whenComplete(() {
showBottomSheetThenCalled = true;
});
await tester.pumpAndSettle();
expect(showBottomSheetThenCalled, isFalse);
expect(find.text('BottomSheet'), findsOneWidget);
// Swipe the bottom sheet, attempting to dismiss it.
await tester.drag(find.text('BottomSheet'), const Offset(0.0, 150.0));
await tester.pumpAndSettle(); // Bottom sheet should not dismiss.
expect(showBottomSheetThenCalled, isFalse);
expect(find.text('BottomSheet'), findsOneWidget);
});
testWidgets('Swiping down a BottomSheet should dismiss it when enableDrag is true', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
bool showBottomSheetThenCalled = false;
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
await tester.pump();
expect(showBottomSheetThenCalled, isFalse);
expect(find.text('BottomSheet'), findsNothing);
scaffoldKey.currentState!.showBottomSheet((BuildContext context) {
return const SizedBox(
height: 200.0,
child: Text('BottomSheet'),
);
},
enableDrag: true
).closed.whenComplete(() {
showBottomSheetThenCalled = true;
});
await tester.pumpAndSettle();
expect(showBottomSheetThenCalled, isFalse);
expect(find.text('BottomSheet'), findsOneWidget);
// Swipe the bottom sheet to dismiss it.
await tester.drag(find.text('BottomSheet'), const Offset(0.0, 150.0));
await tester.pumpAndSettle(); // Bottom sheet dismiss animation.
expect(showBottomSheetThenCalled, isTrue);
expect(find.text('BottomSheet'), findsNothing);
});
testWidgets('Tapping on a BottomSheet should not trigger a rebuild when enableDrag is true', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/126833.
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
int buildCount = 0;
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
await tester.pump();
expect(buildCount, 0);
expect(find.text('BottomSheet'), findsNothing);
scaffoldKey.currentState!.showBottomSheet((BuildContext context) {
buildCount++;
return const SizedBox(
height: 200.0,
child: Text('BottomSheet'),
);
},
enableDrag: true,
);
await tester.pumpAndSettle();
expect(buildCount, 1);
expect(find.text('BottomSheet'), findsOneWidget);
// Tap on bottom sheet should not trigger a rebuild.
await tester.tap(find.text('BottomSheet'));
await tester.pumpAndSettle();
expect(buildCount, 1);
expect(find.text('BottomSheet'), findsOneWidget);
});
testWidgets('Modal BottomSheet builder should only be called once', (WidgetTester tester) async {
late BuildContext savedContext;
await tester.pumpWidget(MaterialApp(
home: Builder(
builder: (BuildContext context) {
savedContext = context;
return Container();
},
),
));
int numBuilderCalls = 0;
showModalBottomSheet<void>(
context: savedContext,
isDismissible: false,
builder: (BuildContext context) {
numBuilderCalls++;
return const Text('BottomSheet');
},
);
await tester.pumpAndSettle();
expect(numBuilderCalls, 1);
// Swipe the bottom sheet to dismiss it.
await tester.drag(find.text('BottomSheet'), const Offset(0.0, 150.0));
await tester.pumpAndSettle(); // Bottom sheet dismiss animation.
expect(numBuilderCalls, 1);
});
testWidgets('Tapping on a modal BottomSheet should not dismiss it', (WidgetTester tester) async {
late BuildContext savedContext;
await tester.pumpWidget(
MaterialApp(
home: Builder(
builder: (BuildContext context) {
savedContext = context;
return Container();
},
),
),
);
await tester.pump();
expect(find.text('BottomSheet'), findsNothing);
bool showBottomSheetThenCalled = false;
showModalBottomSheet<void>(
context: savedContext,
builder: (BuildContext context) => const Text('BottomSheet'),
).then<void>((void value) {
showBottomSheetThenCalled = true;
});
await tester.pumpAndSettle();
expect(find.text('BottomSheet'), findsOneWidget);
expect(showBottomSheetThenCalled, isFalse);
// Tap on the bottom sheet itself, it should not be dismissed
await tester.tap(find.text('BottomSheet'));
await tester.pumpAndSettle();
expect(find.text('BottomSheet'), findsOneWidget);
expect(showBottomSheetThenCalled, isFalse);
});
testWidgets('Tapping outside a modal BottomSheet should dismiss it by default', (WidgetTester tester) async {
late BuildContext savedContext;
await tester.pumpWidget(MaterialApp(
home: Builder(
builder: (BuildContext context) {
savedContext = context;
return Container();
},
),
));
await tester.pump();
expect(find.text('BottomSheet'), findsNothing);
bool showBottomSheetThenCalled = false;
showModalBottomSheet<void>(
context: savedContext,
builder: (BuildContext context) => const Text('BottomSheet'),
).then<void>((void value) {
showBottomSheetThenCalled = true;
});
await tester.pumpAndSettle();
expect(find.text('BottomSheet'), findsOneWidget);
expect(showBottomSheetThenCalled, isFalse);
// Tap above the bottom sheet to dismiss it.
await tester.tapAt(const Offset(20.0, 20.0));
await tester.pumpAndSettle(); // Bottom sheet dismiss animation.
expect(showBottomSheetThenCalled, isTrue);
expect(find.text('BottomSheet'), findsNothing);
});
testWidgets('Tapping outside a modal BottomSheet should dismiss it when isDismissible=true', (WidgetTester tester) async {
late BuildContext savedContext;
await tester.pumpWidget(MaterialApp(
home: Builder(
builder: (BuildContext context) {
savedContext = context;
return Container();
},
),
));
await tester.pump();
expect(find.text('BottomSheet'), findsNothing);
bool showBottomSheetThenCalled = false;
showModalBottomSheet<void>(
context: savedContext,
builder: (BuildContext context) => const Text('BottomSheet'),
).then<void>((void value) {
showBottomSheetThenCalled = true;
});
await tester.pumpAndSettle();
expect(find.text('BottomSheet'), findsOneWidget);
expect(showBottomSheetThenCalled, isFalse);
// Tap above the bottom sheet to dismiss it.
await tester.tapAt(const Offset(20.0, 20.0));
await tester.pumpAndSettle(); // Bottom sheet dismiss animation.
expect(showBottomSheetThenCalled, isTrue);
expect(find.text('BottomSheet'), findsNothing);
});
testWidgets('Verify that the BottomSheet animates non-linearly', (WidgetTester tester) async {
late BuildContext savedContext;
await tester.pumpWidget(MaterialApp(
home: Builder(
builder: (BuildContext context) {
savedContext = context;
return Container();
},
),
));
await tester.pump();
expect(find.text('BottomSheet'), findsNothing);
showModalBottomSheet<void>(
context: savedContext,
builder: (BuildContext context) => const Text('BottomSheet'),
);
await tester.pump();
await checkNonLinearAnimation(tester);
await tester.pumpAndSettle();
// Tap above the bottom sheet to dismiss it.
await tester.tapAt(const Offset(20.0, 20.0));
await tester.pump();
await checkNonLinearAnimation(tester);
await tester.pumpAndSettle(); // Bottom sheet dismiss animation.
expect(find.text('BottomSheet'), findsNothing);
});
// Regression test for https://github.com/flutter/flutter/issues/121098
testWidgets('Verify that accessibleNavigation has no impact on the BottomSheet animation', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
data: const MediaQueryData(accessibleNavigation: true),
child: child!,
);
},
home: const Center(child: Text('Test')),
));
await tester.pump();
expect(find.text('BottomSheet'), findsNothing);
final BuildContext homeContext = tester.element(find.text('Test'));
showModalBottomSheet<void>(
context: homeContext,
builder: (BuildContext context) => const Text('BottomSheet'),
);
await tester.pump();
await checkNonLinearAnimation(tester);
await tester.pumpAndSettle();
});
testWidgets('Tapping outside a modal BottomSheet should not dismiss it when isDismissible=false', (WidgetTester tester) async {
late BuildContext savedContext;
await tester.pumpWidget(
MaterialApp(
home: Builder(
builder: (BuildContext context) {
savedContext = context;
return Container();
},
),
),
);
await tester.pump();
expect(find.text('BottomSheet'), findsNothing);
bool showBottomSheetThenCalled = false;
showModalBottomSheet<void>(
context: savedContext,
builder: (BuildContext context) => const Text('BottomSheet'),
isDismissible: false,
).then<void>((void value) {
showBottomSheetThenCalled = true;
});
await tester.pumpAndSettle();
expect(find.text('BottomSheet'), findsOneWidget);
expect(showBottomSheetThenCalled, isFalse);
// Tap above the bottom sheet, attempting to dismiss it.
await tester.tapAt(const Offset(20.0, 20.0));
await tester.pumpAndSettle(); // Bottom sheet should not dismiss.
expect(showBottomSheetThenCalled, isFalse);
expect(find.text('BottomSheet'), findsOneWidget);
});
testWidgets('Swiping down a modal BottomSheet should dismiss it by default', (WidgetTester tester) async {
late BuildContext savedContext;
await tester.pumpWidget(MaterialApp(
home: Builder(
builder: (BuildContext context) {
savedContext = context;
return Container();
},
),
));
await tester.pump();
expect(find.text('BottomSheet'), findsNothing);
bool showBottomSheetThenCalled = false;
showModalBottomSheet<void>(
context: savedContext,
isDismissible: false,
builder: (BuildContext context) => const Text('BottomSheet'),
).then<void>((void value) {
showBottomSheetThenCalled = true;
});
await tester.pumpAndSettle();
expect(find.text('BottomSheet'), findsOneWidget);
expect(showBottomSheetThenCalled, isFalse);
// Swipe the bottom sheet to dismiss it.
await tester.drag(find.text('BottomSheet'), const Offset(0.0, 150.0));
await tester.pumpAndSettle(); // Bottom sheet dismiss animation.
expect(showBottomSheetThenCalled, isTrue);
expect(find.text('BottomSheet'), findsNothing);
});
testWidgets('Swiping down a modal BottomSheet should not dismiss it when enableDrag is false', (WidgetTester tester) async {
late BuildContext savedContext;
await tester.pumpWidget(MaterialApp(
home: Builder(
builder: (BuildContext context) {
savedContext = context;
return Container();
},
),
));
await tester.pump();
expect(find.text('BottomSheet'), findsNothing);
bool showBottomSheetThenCalled = false;
showModalBottomSheet<void>(
context: savedContext,
isDismissible: false,
enableDrag: false,
builder: (BuildContext context) => const Text('BottomSheet'),
).then<void>((void value) {
showBottomSheetThenCalled = true;
});
await tester.pumpAndSettle();
expect(find.text('BottomSheet'), findsOneWidget);
expect(showBottomSheetThenCalled, isFalse);
// Swipe the bottom sheet, attempting to dismiss it.
await tester.drag(find.text('BottomSheet'), const Offset(0.0, 150.0));
await tester.pumpAndSettle(); // Bottom sheet should not dismiss.
expect(showBottomSheetThenCalled, isFalse);
expect(find.text('BottomSheet'), findsOneWidget);
});
testWidgets('Swiping down a modal BottomSheet should dismiss it when enableDrag is true', (WidgetTester tester) async {
late BuildContext savedContext;
await tester.pumpWidget(MaterialApp(
home: Builder(
builder: (BuildContext context) {
savedContext = context;
return Container();
},
),
));
await tester.pump();
expect(find.text('BottomSheet'), findsNothing);
bool showBottomSheetThenCalled = false;
showModalBottomSheet<void>(
context: savedContext,
isDismissible: false,
builder: (BuildContext context) => const Text('BottomSheet'),
).then<void>((void value) {
showBottomSheetThenCalled = true;
});
await tester.pumpAndSettle();
expect(find.text('BottomSheet'), findsOneWidget);
expect(showBottomSheetThenCalled, isFalse);
// Swipe the bottom sheet to dismiss it.
await tester.drag(find.text('BottomSheet'), const Offset(0.0, 150.0));
await tester.pumpAndSettle(); // Bottom sheet dismiss animation.
expect(showBottomSheetThenCalled, isTrue);
expect(find.text('BottomSheet'), findsNothing);
});
testWidgets('Modal BottomSheet builder should only be called once', (WidgetTester tester) async {
late BuildContext savedContext;
await tester.pumpWidget(MaterialApp(
home: Builder(
builder: (BuildContext context) {
savedContext = context;
return Container();
},
),
));
int numBuilderCalls = 0;
showModalBottomSheet<void>(
context: savedContext,
isDismissible: false,
builder: (BuildContext context) {
numBuilderCalls++;
return const Text('BottomSheet');
},
);
await tester.pumpAndSettle();
expect(numBuilderCalls, 1);
// Swipe the bottom sheet to dismiss it.
await tester.drag(find.text('BottomSheet'), const Offset(0.0, 150.0));
await tester.pumpAndSettle(); // Bottom sheet dismiss animation.
expect(numBuilderCalls, 1);
});
testWidgets('Verify that a downwards fling dismisses a persistent BottomSheet', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
bool showBottomSheetThenCalled = false;
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
expect(showBottomSheetThenCalled, isFalse);
expect(find.text('BottomSheet'), findsNothing);
scaffoldKey.currentState!.showBottomSheet((BuildContext context) {
return Container(
margin: const EdgeInsets.all(40.0),
child: const Text('BottomSheet'),
);
}).closed.whenComplete(() {
showBottomSheetThenCalled = true;
});
expect(showBottomSheetThenCalled, isFalse);
expect(find.text('BottomSheet'), findsNothing);
await tester.pump(); // bottom sheet show animation starts
expect(showBottomSheetThenCalled, isFalse);
expect(find.text('BottomSheet'), findsOneWidget);
await tester.pump(const Duration(seconds: 1)); // animation done
expect(showBottomSheetThenCalled, isFalse);
expect(find.text('BottomSheet'), findsOneWidget);
// The fling below must be such that the velocity estimation examines an
// offset greater than the kTouchSlop. Too slow or too short a distance, and
// it won't trigger. Also, it must not be so much that it drags the bottom
// sheet off the screen, or we won't see it after we pump!
await tester.fling(find.text('BottomSheet'), const Offset(0.0, 50.0), 2000.0);
await tester.pump(); // drain the microtask queue (Future completion callback)
expect(showBottomSheetThenCalled, isTrue);
expect(find.text('BottomSheet'), findsOneWidget);
await tester.pump(); // bottom sheet dismiss animation starts
expect(showBottomSheetThenCalled, isTrue);
expect(find.text('BottomSheet'), findsOneWidget);
await tester.pump(const Duration(seconds: 1)); // animation done
expect(showBottomSheetThenCalled, isTrue);
expect(find.text('BottomSheet'), findsNothing);
});
testWidgets('Verify that dragging past the bottom dismisses a persistent BottomSheet', (WidgetTester tester) async {
// This is a regression test for https://github.com/flutter/flutter/issues/5528
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
scaffoldKey.currentState!.showBottomSheet((BuildContext context) {
return Container(
margin: const EdgeInsets.all(40.0),
child: const Text('BottomSheet'),
);
});
await tester.pump(); // bottom sheet show animation starts
await tester.pump(const Duration(seconds: 1)); // animation done
expect(find.text('BottomSheet'), findsOneWidget);
await tester.fling(find.text('BottomSheet'), const Offset(0.0, 400.0), 1000.0);
await tester.pump(); // drain the microtask queue (Future completion callback)
await tester.pump(); // bottom sheet dismiss animation starts
await tester.pump(const Duration(seconds: 1)); // animation done
expect(find.text('BottomSheet'), findsNothing);
});
testWidgets('modal BottomSheet has no top MediaQuery', (WidgetTester tester) async {
late BuildContext outerContext;
late BuildContext innerContext;
await tester.pumpWidget(Localizations(
locale: const Locale('en', 'US'),
delegates: const <LocalizationsDelegate<dynamic>>[
DefaultWidgetsLocalizations.delegate,
DefaultMaterialLocalizations.delegate,
],
child: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(
padding: EdgeInsets.all(50.0),
size: Size(400.0, 600.0),
),
child: Navigator(
onGenerateRoute: (_) {
return PageRouteBuilder<void>(
pageBuilder: (BuildContext context, Animation<double> animation, Animation<double> secondaryAnimation) {
outerContext = context;
return Container();
},
);
},
),
),
),
));
showModalBottomSheet<void>(
context: outerContext,
builder: (BuildContext context) {
innerContext = context;
return Container();
},
);
await tester.pump();
await tester.pump(const Duration(seconds: 1));
expect(
MediaQuery.of(outerContext).padding,
const EdgeInsets.all(50.0),
);
expect(
MediaQuery.of(innerContext).padding,
const EdgeInsets.only(left: 50.0, right: 50.0, bottom: 50.0),
);
});
testWidgets('modal BottomSheet can insert a SafeArea', (WidgetTester tester) async {
late BuildContext outerContext;
late BuildContext innerContext;
await tester.pumpWidget(Localizations(
locale: const Locale('en', 'US'),
delegates: const <LocalizationsDelegate<dynamic>>[
DefaultWidgetsLocalizations.delegate,
DefaultMaterialLocalizations.delegate,
],
child: Directionality(
textDirection: TextDirection.ltr,
child: MediaQuery(
data: const MediaQueryData(
padding: EdgeInsets.all(50.0),
size: Size(400.0, 600.0),
),
child: Navigator(
onGenerateRoute: (_) {
return PageRouteBuilder<void>(
pageBuilder: (BuildContext context, Animation<double> animation, Animation<double> secondaryAnimation) {
outerContext = context;
return Container();
},
);
},
),
),
),
));
// Without a SafeArea (useSafeArea is false by default)
showModalBottomSheet<void>(
context: outerContext,
builder: (BuildContext context) {
innerContext = context;
return Container();
},
);
await tester.pump();
await tester.pump(const Duration(seconds: 1));
// Top padding is consumed and there is no SafeArea
expect(MediaQuery.of(innerContext).padding.top, 0);
expect(find.byType(SafeArea), findsNothing);
// With a SafeArea
showModalBottomSheet<void>(
context: outerContext,
useSafeArea: true,
builder: (BuildContext context) {
innerContext = context;
return Container();
},
);
await tester.pump();
await tester.pump(const Duration(seconds: 1));
// A SafeArea is inserted, with left / top / right true but bottom false.
final Finder safeAreaWidgetFinder = find.byType(SafeArea);
expect(safeAreaWidgetFinder, findsOneWidget);
final SafeArea safeAreaWidget = safeAreaWidgetFinder.evaluate().single.widget as SafeArea;
expect(safeAreaWidget.left, true);
expect(safeAreaWidget.top, true);
expect(safeAreaWidget.right, true);
expect(safeAreaWidget.bottom, false);
// Because that SafeArea is inserted, no left / top / right padding remains
// for `builder` to consume. Bottom padding does remain.
expect(MediaQuery.of(innerContext).padding, const EdgeInsets.fromLTRB(0, 0, 0, 50.0));
});
testWidgets('modal BottomSheet has semantics', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
showModalBottomSheet<void>(context: scaffoldKey.currentContext!, builder: (BuildContext context) {
return const Text('BottomSheet');
});
await tester.pump(); // bottom sheet show animation starts
await tester.pump(const Duration(seconds: 1)); // animation done
expect(semantics, hasSemantics(TestSemantics.root(
children: <TestSemantics>[
TestSemantics.rootChild(
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
label: 'Dialog',
textDirection: TextDirection.ltr,
flags: <SemanticsFlag>[
SemanticsFlag.scopesRoute,
SemanticsFlag.namesRoute,
],
children: <TestSemantics>[
TestSemantics(
label: 'BottomSheet',
textDirection: TextDirection.ltr,
),
],
),
],
),
TestSemantics(
children: <TestSemantics>[
TestSemantics(
actions: <SemanticsAction>[SemanticsAction.tap, SemanticsAction.dismiss],
label: 'Scrim',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
), ignoreTransform: true, ignoreRect: true, ignoreId: true));
semantics.dispose();
});
testWidgets('Verify that visual properties are passed through', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
const Color color = Colors.pink;
const double elevation = 9.0;
const ShapeBorder shape = BeveledRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(12)));
const Clip clipBehavior = Clip.antiAlias;
const Color barrierColor = Colors.red;
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
showModalBottomSheet<void>(
context: scaffoldKey.currentContext!,
backgroundColor: color,
barrierColor: barrierColor,
elevation: elevation,
shape: shape,
clipBehavior: clipBehavior,
builder: (BuildContext context) {
return const Text('BottomSheet');
},
);
await tester.pump();
await tester.pump(const Duration(seconds: 1));
final BottomSheet bottomSheet = tester.widget(find.byType(BottomSheet));
expect(bottomSheet.backgroundColor, color);
expect(bottomSheet.elevation, elevation);
expect(bottomSheet.shape, shape);
expect(bottomSheet.clipBehavior, clipBehavior);
final ModalBarrier modalBarrier = tester.widget(find.byType(ModalBarrier).last);
expect(modalBarrier.color, barrierColor);
});
testWidgets('Material3 - BottomSheet uses fallback values',
(WidgetTester tester) async {
const Color surfaceColor = Colors.pink;
const Color surfaceTintColor = Colors.blue;
const ShapeBorder defaultShape = RoundedRectangleBorder(
borderRadius: BorderRadius.vertical(
top: Radius.circular(28.0),
));
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
colorScheme: const ColorScheme.light(
surface: surfaceColor,
surfaceTint: surfaceTintColor,
),
useMaterial3: true,
),
home: Scaffold(
body: BottomSheet(
onClosing: () {},
builder: (BuildContext context) {
return Container();
},
),
),
));
final Finder finder = find.descendant(
of: find.byType(BottomSheet),
matching: find.byType(Material),
);
final Material material = tester.widget<Material>(finder);
expect(material.color, surfaceColor);
// Surface tint is no longer used by default.
expect(material.surfaceTintColor, Colors.transparent);
expect(material.elevation, 1.0);
expect(material.shape, defaultShape);
expect(tester.getSize(finder).width, 640);
});
testWidgets('Material3 - BottomSheet has transparent shadow', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
useMaterial3: true,
),
home: Scaffold(
body: BottomSheet(
onClosing: () {},
builder: (BuildContext context) {
return Container();
},
),
),
));
final Material material = tester.widget<Material>(
find.descendant(
of: find.byType(BottomSheet),
matching: find.byType(Material),
),
);
expect(material.shadowColor, Colors.transparent);
});
testWidgets('Material2 - Modal BottomSheet with ScrollController has semantics', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
showModalBottomSheet<void>(
context: scaffoldKey.currentContext!,
builder: (BuildContext context) {
return DraggableScrollableSheet(
expand: false,
builder: (_, ScrollController controller) {
return SingleChildScrollView(
controller: controller,
child: const Text('BottomSheet'),
);
},
);
},
);
await tester.pump(); // bottom sheet show animation starts
await tester.pump(const Duration(seconds: 1)); // animation done
expect(semantics, hasSemantics(TestSemantics.root(
children: <TestSemantics>[
TestSemantics.rootChild(
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
label: 'Dialog',
textDirection: TextDirection.ltr,
flags: <SemanticsFlag>[
SemanticsFlag.scopesRoute,
SemanticsFlag.namesRoute,
],
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.hasImplicitScrolling],
children: <TestSemantics>[
TestSemantics(
label: 'BottomSheet',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
TestSemantics(
children: <TestSemantics>[
TestSemantics(
actions: <SemanticsAction>[SemanticsAction.tap, SemanticsAction.dismiss],
label: 'Scrim',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
), ignoreTransform: true, ignoreRect: true, ignoreId: true));
semantics.dispose();
});
testWidgets('Material3 - Modal BottomSheet with ScrollController has semantics', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
showModalBottomSheet<void>(
context: scaffoldKey.currentContext!,
builder: (BuildContext context) {
return DraggableScrollableSheet(
expand: false,
builder: (_, ScrollController controller) {
return SingleChildScrollView(
controller: controller,
child: const Text('BottomSheet'),
);
},
);
},
);
await tester.pump(); // bottom sheet show animation starts
await tester.pump(const Duration(seconds: 1)); // animation done
expect(semantics, hasSemantics(TestSemantics.root(
children: <TestSemantics>[
TestSemantics.rootChild(
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
label: 'Dialog',
textDirection: TextDirection.ltr,
flags: <SemanticsFlag>[
SemanticsFlag.scopesRoute,
SemanticsFlag.namesRoute,
],
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.hasImplicitScrolling],
children: <TestSemantics>[
TestSemantics(
label: 'BottomSheet',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
),
TestSemantics(
children: <TestSemantics>[
TestSemantics(
actions: <SemanticsAction>[SemanticsAction.tap, SemanticsAction.dismiss],
label: 'Scrim',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
), ignoreTransform: true, ignoreRect: true, ignoreId: true));
semantics.dispose();
});
testWidgets('Material3 - Modal BottomSheet with drag handle has semantics', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
showModalBottomSheet<void>(
context: scaffoldKey.currentContext!,
showDragHandle: true,
builder: (BuildContext context) {
return const Text('BottomSheet');
},
);
await tester.pump(); // bottom sheet show animation starts
await tester.pump(const Duration(seconds: 1)); // animation done
expect(semantics, hasSemantics(TestSemantics.root(
children: <TestSemantics>[
TestSemantics.rootChild(
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
label: 'Dialog',
textDirection: TextDirection.ltr,
flags: <SemanticsFlag>[
SemanticsFlag.scopesRoute,
SemanticsFlag.namesRoute,
],
children: <TestSemantics>[
TestSemantics(
label: 'BottomSheet',
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
actions: <SemanticsAction>[SemanticsAction.tap],
label: 'Dismiss',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
TestSemantics(
children: <TestSemantics>[
TestSemantics(
actions: <SemanticsAction>[SemanticsAction.tap, SemanticsAction.dismiss],
label: 'Scrim',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
), ignoreTransform: true, ignoreRect: true, ignoreId: true));
semantics.dispose();
});
testWidgets('Drag handle color can take MaterialStateProperty', (WidgetTester tester) async {
const Color defaultColor=Colors.blue;
const Color hoveringColor=Colors.green;
Future<void> checkDragHandleAndColors() async {
await tester.pump(); // bottom sheet show animation starts
await tester.pump(const Duration(seconds: 1)); // animation done
final Finder dragHandle = find.bySemanticsLabel('Dismiss');
expect(
tester.getSize(dragHandle),
const Size(48, 48),
);
final Offset center = tester.getCenter(dragHandle);
final Offset edge = tester.getTopLeft(dragHandle) - const Offset(1, 1);
// Shows default drag handle color
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse, pointer: 1);
await gesture.addPointer(location: edge);
await tester.pump();
BoxDecoration boxDecoration=tester.widget<Container>(find.descendant(
of: dragHandle,
matching: find.byWidgetPredicate((Widget widget) => widget is Container && widget.decoration != null),
)).decoration! as BoxDecoration;
expect(boxDecoration.color, defaultColor);
// Shows hovering drag handle color
await gesture.moveTo(center);
await tester.pump();
boxDecoration = tester.widget<Container>(find.descendant(
of: dragHandle,
matching: find.byWidgetPredicate((Widget widget) => widget is Container && widget.decoration != null),
)).decoration! as BoxDecoration;
expect(boxDecoration.color, hoveringColor);
await gesture.removePointer();
}
Widget buildScaffold(GlobalKey scaffoldKey) {
return MaterialApp(
theme: ThemeData.light().copyWith(
bottomSheetTheme: BottomSheetThemeData(
dragHandleColor: MaterialStateColor.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.hovered)) {
return hoveringColor;
}
return defaultColor;
}),
),
),
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
);
}
GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(buildScaffold(scaffoldKey));
showModalBottomSheet<void>(
context: scaffoldKey.currentContext!,
showDragHandle: true,
builder: (BuildContext context) {
return const Text('BottomSheet');
},
);
await checkDragHandleAndColors();
await tester.pumpWidget(Container()); // Reset
scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(buildScaffold(scaffoldKey));
scaffoldKey.currentState!.showBottomSheet((_) {
return Builder(
builder: (BuildContext context) {
return const SizedBox(
height: 200.0,
child: Text('Bottom Sheet'),
);
},
);
}, showDragHandle: true);
await checkDragHandleAndColors();
});
testWidgets('showModalBottomSheet does not use root Navigator by default', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Navigator(onGenerateRoute: (RouteSettings settings) => MaterialPageRoute<void>(builder: (_) {
return const _TestPage();
})),
bottomNavigationBar: BottomNavigationBar(
items: const <BottomNavigationBarItem>[
BottomNavigationBarItem(
icon: Icon(Icons.ac_unit),
label: 'Item 1',
),
BottomNavigationBarItem(
icon: Icon(Icons.style),
label: 'Item 2',
),
],
),
),
));
await tester.tap(find.text('Show bottom sheet'));
await tester.pumpAndSettle();
// Bottom sheet is displayed in correct position within the inner navigator
// and above the BottomNavigationBar.
final double tabBarHeight = tester.getSize(find.byType(BottomNavigationBar)).height;
expect(tester.getBottomLeft(find.byType(BottomSheet)).dy, 600 - tabBarHeight);
});
testWidgets('showModalBottomSheet uses root Navigator when specified', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Navigator(onGenerateRoute: (RouteSettings settings) => MaterialPageRoute<void>(builder: (_) {
return const _TestPage(useRootNavigator: true);
})),
bottomNavigationBar: BottomNavigationBar(
items: const <BottomNavigationBarItem>[
BottomNavigationBarItem(
icon: Icon(Icons.ac_unit),
label: 'Item 1',
),
BottomNavigationBarItem(
icon: Icon(Icons.style),
label: 'Item 2',
),
],
),
),
));
await tester.tap(find.text('Show bottom sheet'));
await tester.pumpAndSettle();
// Bottom sheet is displayed in correct position above all content including
// the BottomNavigationBar.
expect(tester.getBottomLeft(find.byType(BottomSheet)).dy, 600.0);
});
testWidgets('Verify that route settings can be set in the showModalBottomSheet', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
const RouteSettings routeSettings = RouteSettings(name: 'route_name', arguments: 'route_argument');
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
late RouteSettings retrievedRouteSettings;
showModalBottomSheet<void>(
context: scaffoldKey.currentContext!,
routeSettings: routeSettings,
builder: (BuildContext context) {
retrievedRouteSettings = ModalRoute.of(context)!.settings;
return const Text('BottomSheet');
},
);
await tester.pump();
await tester.pump(const Duration(seconds: 1));
expect(retrievedRouteSettings, routeSettings);
});
testWidgets('Verify showModalBottomSheet use AnimationController if provided.', (WidgetTester tester) async {
const Key tapTarget = Key('tap-target');
final AnimationController controller = AnimationController(
vsync: const TestVSync(),
duration: const Duration(seconds: 2),
reverseDuration: const Duration(seconds: 2),
);
addTearDown(controller.dispose);
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
key: tapTarget,
onTap: () {
showModalBottomSheet<void>(
context: context,
// The default duration and reverseDuration is 1 second
transitionAnimationController: controller,
builder: (BuildContext context) {
return const Text('BottomSheet');
},
);
},
behavior: HitTestBehavior.opaque,
child: const SizedBox(
height: 100.0,
width: 100.0,
),
);
},
),
),
));
expect(find.text('BottomSheet'), findsNothing);
await tester.tap(find.byKey(tapTarget)); // Opening animation will start after tapping
await tester.pump();
expect(find.text('BottomSheet'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 2000));
expect(find.text('BottomSheet'), findsOneWidget);
// Tapping above the bottom sheet to dismiss it.
await tester.tapAt(const Offset(20.0, 20.0)); // Closing animation will start after tapping
await tester.pump();
expect(find.text('BottomSheet'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 2000));
// The bottom sheet should still be present at the very end of the animation.
expect(find.text('BottomSheet'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 1));
// The bottom sheet should not be showing any longer.
expect(find.text('BottomSheet'), findsNothing);
});
// Regression test for https://github.com/flutter/flutter/issues/87592
testWidgets('the framework do not dispose the transitionAnimationController provided by user.', (WidgetTester tester) async {
const Key tapTarget = Key('tap-target');
final AnimationController controller = AnimationController(
vsync: const TestVSync(),
duration: const Duration(seconds: 2),
reverseDuration: const Duration(seconds: 2),
);
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
key: tapTarget,
onTap: () {
showModalBottomSheet<void>(
context: context,
// The default duration and reverseDuration is 1 second
transitionAnimationController: controller,
builder: (BuildContext context) {
return const Text('BottomSheet');
},
);
},
behavior: HitTestBehavior.opaque,
child: const SizedBox(
height: 100.0,
width: 100.0,
),
);
},
),
),
));
expect(find.text('BottomSheet'), findsNothing);
await tester.tap(find.byKey(tapTarget)); // Opening animation will start after tapping
await tester.pump();
expect(find.text('BottomSheet'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 2000));
expect(find.text('BottomSheet'), findsOneWidget);
// Tapping above the bottom sheet to dismiss it.
await tester.tapAt(const Offset(20.0, 20.0)); // Closing animation will start after tapping
await tester.pump();
expect(find.text('BottomSheet'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 2000));
// The bottom sheet should still be present at the very end of the animation.
expect(find.text('BottomSheet'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 1));
// The bottom sheet should not be showing any longer.
expect(find.text('BottomSheet'), findsNothing);
controller.dispose();
// Double disposal will throw.
expect(tester.takeException(), isNull);
});
testWidgets('Verify persistence BottomSheet use AnimationController if provided.', (WidgetTester tester) async {
const Key tapTarget = Key('tap-target');
const Key tapTargetToClose = Key('tap-target-to-close');
final AnimationController controller = AnimationController(
vsync: const TestVSync(),
duration: const Duration(seconds: 2),
reverseDuration: const Duration(seconds: 2),
);
addTearDown(controller.dispose);
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
key: tapTarget,
onTap: () {
showBottomSheet(
context: context,
// The default duration and reverseDuration is 1 second
transitionAnimationController: controller,
builder: (BuildContext context) {
return ElevatedButton(
key: tapTargetToClose,
onPressed: () => Navigator.pop(context),
child: const Text('BottomSheet'),
);
},
);
},
behavior: HitTestBehavior.opaque,
child: const SizedBox(
height: 100.0,
width: 100.0,
),
);
},
),
),
));
expect(find.text('BottomSheet'), findsNothing);
await tester.tap(find.byKey(tapTarget)); // Opening animation will start after tapping
await tester.pump();
expect(find.text('BottomSheet'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 2000));
expect(find.text('BottomSheet'), findsOneWidget);
// Tapping button on the bottom sheet to dismiss it.
await tester.tap(find.byKey(tapTargetToClose)); // Closing animation will start after tapping
await tester.pump();
expect(find.text('BottomSheet'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 2000));
// The bottom sheet should still be present at the very end of the animation.
expect(find.text('BottomSheet'), findsOneWidget);
await tester.pump(const Duration(milliseconds: 1));
// The bottom sheet should not be showing any longer.
expect(find.text('BottomSheet'), findsNothing);
});
// Regression test for https://github.com/flutter/flutter/issues/87708
testWidgets('Each of the internal animation controllers should be disposed by the framework.', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey();
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
scaffoldKey.currentState!.showBottomSheet((_) {
return Builder(
builder: (BuildContext context) {
return Container(height: 200.0);
},
);
});
await tester.pump();
expect(find.byType(BottomSheet), findsOneWidget);
// The first sheet's animation is still running.
// Trigger the second sheet will remove the first sheet from tree.
scaffoldKey.currentState!.showBottomSheet((_) {
return Builder(
builder: (BuildContext context) {
return Container(height: 200.0);
},
);
});
await tester.pump();
expect(find.byType(BottomSheet), findsOneWidget);
// Remove the Scaffold from the tree.
await tester.pumpWidget(const SizedBox.shrink());
// If the internal animation controller do not dispose will throw
// FlutterError:<ScaffoldState#1981a(tickers: tracking 1 ticker) was disposed with an active
// Ticker.
expect(tester.takeException(), isNull);
});
// Regression test for https://github.com/flutter/flutter/issues/99627
testWidgets('The old route entry should be removed when a new sheet popup', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey();
PersistentBottomSheetController? sheetController;
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
final ModalRoute<dynamic> route = ModalRoute.of(scaffoldKey.currentContext!)!;
expect(route.canPop, false);
scaffoldKey.currentState!.showBottomSheet((_) {
return Builder(
builder: (BuildContext context) {
return Container(height: 200.0);
},
);
});
await tester.pump();
expect(find.byType(BottomSheet), findsOneWidget);
expect(route.canPop, true);
// Trigger the second sheet will remove the first sheet from tree.
sheetController = scaffoldKey.currentState!.showBottomSheet((_) {
return Builder(
builder: (BuildContext context) {
return Container(height: 200.0);
},
);
});
await tester.pump();
expect(find.byType(BottomSheet), findsOneWidget);
expect(route.canPop, true);
sheetController.close();
expect(route.canPop, false);
});
// Regression test for https://github.com/flutter/flutter/issues/87708
testWidgets('The framework does not dispose of the transitionAnimationController provided by user.', (WidgetTester tester) async {
const Key tapTarget = Key('tap-target');
const Key tapTargetToClose = Key('tap-target-to-close');
final AnimationController controller = AnimationController(
vsync: const TestVSync(),
duration: const Duration(seconds: 2),
reverseDuration: const Duration(seconds: 2),
);
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Builder(
builder: (BuildContext context) {
return GestureDetector(
key: tapTarget,
onTap: () {
showBottomSheet(
context: context,
transitionAnimationController: controller,
builder: (BuildContext context) {
return ElevatedButton(
key: tapTargetToClose,
onPressed: () => Navigator.pop(context),
child: const Text('BottomSheet'),
);
},
);
},
behavior: HitTestBehavior.opaque,
child: const SizedBox(
height: 100.0,
width: 100.0,
),
);
},
),
),
));
expect(find.text('BottomSheet'), findsNothing);
await tester.tap(find.byKey(tapTarget)); // Open the sheet.
await tester.pumpAndSettle(); // Finish the animation.
expect(find.text('BottomSheet'), findsOneWidget);
// Tapping button on the bottom sheet to dismiss it.
await tester.tap(find.byKey(tapTargetToClose)); // Closing the sheet.
await tester.pumpAndSettle(); // Finish the animation.
expect(find.text('BottomSheet'), findsNothing);
await tester.pumpWidget(const SizedBox.shrink());
controller.dispose();
// Double dispose will throw.
expect(tester.takeException(), isNull);
});
testWidgets('Calling PersistentBottomSheetController.close does not crash when it is not the current bottom sheet', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/93717
PersistentBottomSheetController? sheetController1;
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Builder(builder: (BuildContext context) {
return SafeArea(
child: Column(
children: <Widget>[
ElevatedButton(
child: const Text('show 1'),
onPressed: () {
sheetController1 = Scaffold.of(context).showBottomSheet(
(BuildContext context) => const Text('BottomSheet 1'),
);
},
),
ElevatedButton(
child: const Text('show 2'),
onPressed: () {
Scaffold.of(context).showBottomSheet(
(BuildContext context) => const Text('BottomSheet 2'),
);
},
),
ElevatedButton(
child: const Text('close 1'),
onPressed: (){
sheetController1!.close();
},
),
],
),
);
}),
),
));
await tester.tap(find.text('show 1'));
await tester.pumpAndSettle();
expect(find.text('BottomSheet 1'), findsOneWidget);
await tester.tap(find.text('show 2'));
await tester.pumpAndSettle();
expect(find.text('BottomSheet 2'), findsOneWidget);
// This will throw an assertion if regressed
await tester.tap(find.text('close 1'));
await tester.pumpAndSettle();
expect(find.text('BottomSheet 2'), findsOneWidget);
});
testWidgets('ModalBottomSheetRoute shows BottomSheet correctly', (WidgetTester tester) async {
late BuildContext savedContext;
await tester.pumpWidget(
MaterialApp(
home: Builder(
builder: (BuildContext context) {
savedContext = context;
return Container();
},
),
),
);
await tester.pump();
expect(find.byType(BottomSheet), findsNothing);
// Bring up bottom sheet.
final NavigatorState navigator = Navigator.of(savedContext);
navigator.push(
ModalBottomSheetRoute<void>(
isScrollControlled: false,
builder: (BuildContext context) => Container(),
),
);
await tester.pumpAndSettle();
expect(find.byType(BottomSheet), findsOneWidget);
});
group('Modal BottomSheet avoids overlapping display features', () {
testWidgets('positioning using anchorPoint', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: child!,
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
showModalBottomSheet<void>(
context: context,
builder: (BuildContext context) {
return const Placeholder();
},
anchorPoint: const Offset(1000, 0),
);
await tester.pumpAndSettle();
// Should take the right side of the screen
expect(tester.getTopLeft(find.byType(Placeholder)).dx, 410);
expect(tester.getBottomRight(find.byType(Placeholder)).dx, 800);
});
testWidgets('positioning using Directionality', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: Directionality(
textDirection: TextDirection.rtl,
child: child!,
),
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
showModalBottomSheet<void>(
context: context,
builder: (BuildContext context) {
return const Placeholder();
},
);
await tester.pumpAndSettle();
// This is RTL, so it should place the dialog on the right screen
expect(tester.getTopLeft(find.byType(Placeholder)).dx, 410);
expect(tester.getBottomRight(find.byType(Placeholder)).dx, 800);
});
testWidgets('default positioning', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
builder: (BuildContext context, Widget? child) {
return MediaQuery(
// Display has a vertical hinge down the middle
data: const MediaQueryData(
size: Size(800, 600),
displayFeatures: <DisplayFeature>[
DisplayFeature(
bounds: Rect.fromLTRB(390, 0, 410, 600),
type: DisplayFeatureType.hinge,
state: DisplayFeatureState.unknown,
),
],
),
child: child!,
);
},
home: const Center(child: Text('Test')),
),
);
final BuildContext context = tester.element(find.text('Test'));
showModalBottomSheet<void>(
context: context,
builder: (BuildContext context) {
return const Placeholder();
},
);
await tester.pumpAndSettle();
// By default it should place the dialog on the left screen
expect(tester.getTopLeft(find.byType(Placeholder)).dx, 0.0);
expect(tester.getBottomRight(find.byType(Placeholder)).dx, 390.0);
});
});
group('constraints', () {
testWidgets('Material3 - Default constraints are max width 640', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const MediaQuery(
data: MediaQueryData(size: Size(1000, 1000)),
child: Scaffold(
body: Center(child: Text('body')),
bottomSheet: Placeholder(fallbackWidth: 800),
),
),
),
);
expect(tester.getSize(find.byType(Placeholder)).width, 640);
});
testWidgets('Material2 - No constraints by default for bottomSheet property', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
// This test is specific to Material2 because Material3 sets constraints by default for BottomSheet.
theme: ThemeData(useMaterial3: false),
home: const Scaffold(
body: Center(child: Text('body')),
bottomSheet: Text('BottomSheet'),
),
));
expect(find.text('BottomSheet'), findsOneWidget);
expect(
tester.getRect(find.text('BottomSheet')),
const Rect.fromLTRB(0, 586, 154, 600),
);
});
testWidgets('No constraints by default for showBottomSheet', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
// This test is specific to Material2 because Material3 sets constraints by default for BottomSheet.
theme: ThemeData(useMaterial3: false),
home: Scaffold(
body: Builder(builder: (BuildContext context) {
return Center(
child: ElevatedButton(
child: const Text('Press me'),
onPressed: () {
Scaffold.of(context).showBottomSheet(
(BuildContext context) => const Text('BottomSheet'),
);
},
),
);
}),
),
));
expect(find.text('BottomSheet'), findsNothing);
await tester.tap(find.text('Press me'));
await tester.pumpAndSettle();
expect(find.text('BottomSheet'), findsOneWidget);
expect(
tester.getRect(find.text('BottomSheet')),
const Rect.fromLTRB(0, 586, 154, 600),
);
});
testWidgets('No constraints by default for showModalBottomSheet', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
// This test is specific to Material2 because Material3 sets constraints by default for BottomSheet.
theme: ThemeData(useMaterial3: false),
home: Scaffold(
body: Builder(builder: (BuildContext context) {
return Center(
child: ElevatedButton(
child: const Text('Press me'),
onPressed: () {
showModalBottomSheet<void>(
context: context,
builder: (BuildContext context) => const Text('BottomSheet'),
);
},
),
);
}),
),
));
expect(find.text('BottomSheet'), findsNothing);
await tester.tap(find.text('Press me'));
await tester.pumpAndSettle();
expect(find.text('BottomSheet'), findsOneWidget);
expect(
tester.getRect(find.text('BottomSheet')),
const Rect.fromLTRB(0, 586, 800, 600),
);
});
testWidgets('Material3 - Theme constraints used for bottomSheet property', (WidgetTester tester) async {
const double sheetMaxWidth = 80.0;
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
useMaterial3: true,
bottomSheetTheme: const BottomSheetThemeData(
constraints: BoxConstraints(maxWidth: sheetMaxWidth),
),
),
home: Scaffold(
body: const Center(child: Text('body')),
bottomSheet: const Text('BottomSheet'),
floatingActionButton: FloatingActionButton(onPressed: () {}, child: const Icon(Icons.add)),
),
));
expect(find.text('BottomSheet'), findsOneWidget);
// Should be centered and only 80dp wide.
final Rect bottomSheetRect = tester.getRect(find.text('BottomSheet'));
expect(bottomSheetRect.left, 800 / 2 - sheetMaxWidth / 2);
expect(bottomSheetRect.width, sheetMaxWidth);
// Ensure the FAB is overlapping the top of the sheet.
expect(find.byIcon(Icons.add), findsOneWidget);
final Rect iconRect = tester.getRect(find.byIcon(Icons.add));
expect(iconRect.top, bottomSheetRect.top - iconRect.height / 2);
});
testWidgets('Material2 - Theme constraints used for bottomSheet property', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
useMaterial3: false,
bottomSheetTheme: const BottomSheetThemeData(
constraints: BoxConstraints(maxWidth: 80),
),
),
home: Scaffold(
body: const Center(child: Text('body')),
bottomSheet: const Text('BottomSheet'),
floatingActionButton: FloatingActionButton(onPressed: () {}, child: const Icon(Icons.add)),
),
));
expect(find.text('BottomSheet'), findsOneWidget);
// Should be centered and only 80dp wide
expect(
tester.getRect(find.text('BottomSheet')),
const Rect.fromLTRB(360, 558, 440, 600),
);
// Ensure the FAB is overlapping the top of the sheet
expect(find.byIcon(Icons.add), findsOneWidget);
expect(
tester.getRect(find.byIcon(Icons.add)),
const Rect.fromLTRB(744, 544, 768, 568),
);
});
testWidgets('Theme constraints used for showBottomSheet', (WidgetTester tester) async {
const double sheetMaxWidth = 80.0;
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
bottomSheetTheme: const BottomSheetThemeData(
constraints: BoxConstraints(maxWidth: sheetMaxWidth),
),
),
home: Scaffold(
body: Builder(builder: (BuildContext context) {
return Center(
child: ElevatedButton(
child: const Text('Press me'),
onPressed: () {
Scaffold.of(context).showBottomSheet(
(BuildContext context) => const Text('BottomSheet'),
);
},
),
);
}),
),
));
expect(find.text('BottomSheet'), findsNothing);
await tester.tap(find.text('Press me'));
await tester.pumpAndSettle();
expect(find.text('BottomSheet'), findsOneWidget);
// Should be centered and only 80dp wide.
final Rect bottomSheetRect = tester.getRect(find.text('BottomSheet'));
expect(bottomSheetRect.left, 800 / 2 - sheetMaxWidth / 2);
expect(bottomSheetRect.width, sheetMaxWidth);
});
testWidgets('Theme constraints used for showModalBottomSheet', (WidgetTester tester) async {
const double sheetMaxWidth = 80.0;
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
bottomSheetTheme: const BottomSheetThemeData(
constraints: BoxConstraints(maxWidth: sheetMaxWidth),
),
),
home: Scaffold(
body: Builder(builder: (BuildContext context) {
return Center(
child: ElevatedButton(
child: const Text('Press me'),
onPressed: () {
showModalBottomSheet<void>(
context: context,
builder: (BuildContext context) => const Text('BottomSheet'),
);
},
),
);
}),
),
));
expect(find.text('BottomSheet'), findsNothing);
await tester.tap(find.text('Press me'));
await tester.pumpAndSettle();
expect(find.text('BottomSheet'), findsOneWidget);
// Should be centered and only 80dp wide.
final Rect bottomSheetRect = tester.getRect(find.text('BottomSheet'));
expect(bottomSheetRect.left, 800 / 2 - sheetMaxWidth / 2);
expect(bottomSheetRect.width, sheetMaxWidth);
});
testWidgets('constraints param overrides theme for showBottomSheet', (WidgetTester tester) async {
const double sheetMaxWidth = 100.0;
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
bottomSheetTheme: const BottomSheetThemeData(
constraints: BoxConstraints(maxWidth: 80),
),
),
home: Scaffold(
body: Builder(builder: (BuildContext context) {
return Center(
child: ElevatedButton(
child: const Text('Press me'),
onPressed: () {
Scaffold.of(context).showBottomSheet(
(BuildContext context) => const Text('BottomSheet'),
constraints: const BoxConstraints(maxWidth: sheetMaxWidth),
);
},
),
);
}),
),
));
expect(find.text('BottomSheet'), findsNothing);
await tester.tap(find.text('Press me'));
await tester.pumpAndSettle();
expect(find.text('BottomSheet'), findsOneWidget);
// Should be centered and only 80dp wide.
final Rect bottomSheetRect = tester.getRect(find.text('BottomSheet'));
expect(bottomSheetRect.left, 800 / 2 - sheetMaxWidth / 2);
expect(bottomSheetRect.width, sheetMaxWidth);
});
testWidgets('constraints param overrides theme for showModalBottomSheet', (WidgetTester tester) async {
const double sheetMaxWidth = 100.0;
await tester.pumpWidget(MaterialApp(
theme: ThemeData(
bottomSheetTheme: const BottomSheetThemeData(
constraints: BoxConstraints(maxWidth: 80),
),
),
home: Scaffold(
body: Builder(builder: (BuildContext context) {
return Center(
child: ElevatedButton(
child: const Text('Press me'),
onPressed: () {
showModalBottomSheet<void>(
context: context,
builder: (BuildContext context) => const Text('BottomSheet'),
constraints: const BoxConstraints(maxWidth: sheetMaxWidth),
);
},
),
);
}),
),
));
expect(find.text('BottomSheet'), findsNothing);
await tester.tap(find.text('Press me'));
await tester.pumpAndSettle();
expect(find.text('BottomSheet'), findsOneWidget);
// Should be centered and only 80dp wide.
final Rect bottomSheetRect = tester.getRect(find.text('BottomSheet'));
expect(bottomSheetRect.left, 800 / 2 - sheetMaxWidth / 2);
expect(bottomSheetRect.width, sheetMaxWidth);
});
group('scrollControlDisabledMaxHeightRatio', () {
Future<void> test(
WidgetTester tester,
bool isScrollControlled,
double scrollControlDisabledMaxHeightRatio,
) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Builder(builder: (BuildContext context) {
return Center(
child: ElevatedButton(
child: const Text('Press me'),
onPressed: () {
showModalBottomSheet<void>(
context: context,
isScrollControlled: isScrollControlled,
scrollControlDisabledMaxHeightRatio: scrollControlDisabledMaxHeightRatio,
builder: (BuildContext context) => const SizedBox.expand(
child: Text('BottomSheet'),
),
);
},
),
);
}),
),
),
);
await tester.tap(find.text('Press me'));
await tester.pumpAndSettle();
expect(
tester.getRect(find.text('BottomSheet')),
Rect.fromLTRB(
80,
600 * (isScrollControlled ? 0 : (1 - scrollControlDisabledMaxHeightRatio)),
720,
600,
),
);
}
testWidgets('works at 9 / 16', (WidgetTester tester) {
return test(tester, false, 9.0 / 16.0);
});
testWidgets('works at 8 / 16', (WidgetTester tester) {
return test(tester, false, 8.0 / 16.0);
});
testWidgets('works at isScrollControlled', (WidgetTester tester) {
return test(tester, true, 8.0 / 16.0);
});
});
});
group('showModalBottomSheet modalBarrierDismissLabel', () {
testWidgets('Verify that modalBarrierDismissLabel is used if provided',
(WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
const String customLabel = 'custom label';
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
showModalBottomSheet<void>(
barrierLabel: 'custom label',
context: scaffoldKey.currentContext!,
builder: (BuildContext context) {
return const Text('BottomSheet');
},
);
await tester.pump();
await tester.pump(const Duration(seconds: 1));
final ModalBarrier modalBarrier =
tester.widget(find.byType(ModalBarrier).last);
expect(modalBarrier.semanticsLabel, customLabel);
});
testWidgets('Verify that modalBarrierDismissLabel from context is used if barrierLabel is not provided',
(WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
showModalBottomSheet<void>(
context: scaffoldKey.currentContext!,
builder: (BuildContext context) {
return const Text('BottomSheet');
},
);
await tester.pump();
await tester.pump(const Duration(seconds: 1));
final ModalBarrier modalBarrier =
tester.widget(find.byType(ModalBarrier).last);
expect(modalBarrier.semanticsLabel, MaterialLocalizations.of(scaffoldKey.currentContext!).scrimLabel);
});
});
}
class _TestPage extends StatelessWidget {
const _TestPage({this.useRootNavigator});
final bool? useRootNavigator;
@override
Widget build(BuildContext context) {
return Center(
child: TextButton(
child: const Text('Show bottom sheet'),
onPressed: () {
if (useRootNavigator != null) {
showModalBottomSheet<void>(
useRootNavigator: useRootNavigator!,
context: context,
builder: (_) => const Text('Modal bottom sheet'),
);
} else {
showModalBottomSheet<void>(
context: context,
builder: (_) => const Text('Modal bottom sheet'),
);
}
},
),
);
}
}
| flutter/packages/flutter/test/material/bottom_sheet_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/bottom_sheet_test.dart",
"repo_id": "flutter",
"token_count": 33530
} | 688 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:typed_data';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import '../image_data.dart';
void main() {
test('ColorScheme lerp special cases', () {
const ColorScheme scheme = ColorScheme.light();
expect(identical(ColorScheme.lerp(scheme, scheme, 0.5), scheme), true);
});
test('light scheme matches the spec', () {
// Colors should match the Material Design baseline default theme:
// https://material.io/design/color/dark-theme.html#ui-application
// with the new Material 3 colors defaulting to values from the M2
// baseline.
const ColorScheme scheme = ColorScheme.light();
expect(scheme.brightness, Brightness.light);
expect(scheme.primary, const Color(0xff6200ee));
expect(scheme.onPrimary, const Color(0xffffffff));
expect(scheme.primaryContainer, scheme.primary);
expect(scheme.onPrimaryContainer, scheme.onPrimary);
expect(scheme.primaryFixed, scheme.primary);
expect(scheme.primaryFixedDim, scheme.primary);
expect(scheme.onPrimaryFixed, scheme.onPrimary);
expect(scheme.onPrimaryFixedVariant, scheme.onPrimary);
expect(scheme.secondary, const Color(0xff03dac6));
expect(scheme.onSecondary, const Color(0xff000000));
expect(scheme.secondaryContainer, scheme.secondary);
expect(scheme.onSecondaryContainer, scheme.onSecondary);
expect(scheme.secondaryFixed, scheme.secondary);
expect(scheme.secondaryFixedDim, scheme.secondary);
expect(scheme.onSecondaryFixed, scheme.onSecondary);
expect(scheme.onSecondaryFixedVariant, scheme.onSecondary);
expect(scheme.tertiary, scheme.secondary);
expect(scheme.onTertiary, scheme.onSecondary);
expect(scheme.tertiaryContainer, scheme.tertiary);
expect(scheme.onTertiaryContainer, scheme.onTertiary);
expect(scheme.tertiaryFixed, scheme.tertiary);
expect(scheme.tertiaryFixedDim, scheme.tertiary);
expect(scheme.onTertiaryFixed, scheme.onTertiary);
expect(scheme.onTertiaryFixedVariant, scheme.onTertiary);
expect(scheme.error, const Color(0xffb00020));
expect(scheme.onError, const Color(0xffffffff));
expect(scheme.errorContainer, scheme.error);
expect(scheme.onErrorContainer, scheme.onError);
expect(scheme.background, const Color(0xffffffff));
expect(scheme.onBackground, const Color(0xff000000));
expect(scheme.surface, const Color(0xffffffff));
expect(scheme.surfaceBright, scheme.surface);
expect(scheme.surfaceDim, scheme.surface);
expect(scheme.surfaceContainerLowest, scheme.surface);
expect(scheme.surfaceContainerLow, scheme.surface);
expect(scheme.surfaceContainer, scheme.surface);
expect(scheme.surfaceContainerHigh, scheme.surface);
expect(scheme.surfaceContainerHighest, scheme.surface);
expect(scheme.onSurface, const Color(0xff000000));
expect(scheme.surfaceVariant, scheme.surface);
expect(scheme.onSurfaceVariant, scheme.onSurface);
expect(scheme.outline, scheme.onBackground);
expect(scheme.outlineVariant, scheme.onBackground);
expect(scheme.shadow, const Color(0xff000000));
expect(scheme.scrim, const Color(0xff000000));
expect(scheme.inverseSurface, scheme.onSurface);
expect(scheme.onInverseSurface, scheme.surface);
expect(scheme.inversePrimary, scheme.onPrimary);
expect(scheme.surfaceTint, scheme.primary);
});
test('dark scheme matches the spec', () {
// Colors should match the Material Design baseline dark theme:
// https://material.io/design/color/dark-theme.html#ui-application
// with the new Material 3 colors defaulting to values from the M2
// baseline.
const ColorScheme scheme = ColorScheme.dark();
expect(scheme.brightness, Brightness.dark);
expect(scheme.primary, const Color(0xffbb86fc));
expect(scheme.onPrimary, const Color(0xff000000));
expect(scheme.primaryContainer, scheme.primary);
expect(scheme.onPrimaryContainer, scheme.onPrimary);
expect(scheme.primaryFixed, scheme.primary);
expect(scheme.primaryFixedDim, scheme.primary);
expect(scheme.onPrimaryFixed, scheme.onPrimary);
expect(scheme.onPrimaryFixedVariant, scheme.onPrimary);
expect(scheme.secondary, const Color(0xff03dac6));
expect(scheme.onSecondary, const Color(0xff000000));
expect(scheme.secondaryContainer, scheme.secondary);
expect(scheme.onSecondaryContainer, scheme.onSecondary);
expect(scheme.secondaryFixed, scheme.secondary);
expect(scheme.secondaryFixedDim, scheme.secondary);
expect(scheme.onSecondaryFixed, scheme.onSecondary);
expect(scheme.onSecondaryFixedVariant, scheme.onSecondary);
expect(scheme.tertiary, scheme.secondary);
expect(scheme.onTertiary, scheme.onSecondary);
expect(scheme.tertiaryContainer, scheme.tertiary);
expect(scheme.onTertiaryContainer, scheme.onTertiary);
expect(scheme.tertiaryFixed, scheme.tertiary);
expect(scheme.tertiaryFixedDim, scheme.tertiary);
expect(scheme.onTertiaryFixed, scheme.onTertiary);
expect(scheme.onTertiaryFixedVariant, scheme.onTertiary);
expect(scheme.error, const Color(0xffcf6679));
expect(scheme.onError, const Color(0xff000000));
expect(scheme.errorContainer, scheme.error);
expect(scheme.onErrorContainer, scheme.onError);
expect(scheme.background, const Color(0xff121212));
expect(scheme.onBackground, const Color(0xffffffff));
expect(scheme.surface, const Color(0xff121212));
expect(scheme.surfaceBright, scheme.surface);
expect(scheme.surfaceDim, scheme.surface);
expect(scheme.surfaceContainerLowest, scheme.surface);
expect(scheme.surfaceContainerLow, scheme.surface);
expect(scheme.surfaceContainer, scheme.surface);
expect(scheme.surfaceContainerHigh, scheme.surface);
expect(scheme.surfaceContainerHighest, scheme.surface);
expect(scheme.onSurface, const Color(0xffffffff));
expect(scheme.surfaceVariant, scheme.surface);
expect(scheme.onSurfaceVariant, scheme.onSurface);
expect(scheme.outline, scheme.onBackground);
expect(scheme.outlineVariant, scheme.onBackground);
expect(scheme.shadow, const Color(0xff000000));
expect(scheme.scrim, const Color(0xff000000));
expect(scheme.inverseSurface, scheme.onSurface);
expect(scheme.onInverseSurface, scheme.surface);
expect(scheme.inversePrimary, scheme.onPrimary);
expect(scheme.surfaceTint, scheme.primary);
});
test('high contrast light scheme matches the spec', () {
// Colors are based off of the Material Design baseline default theme:
// https://material.io/design/color/dark-theme.html#ui-application
// with the new Material 3 colors defaulting to values from the M2
// baseline.
const ColorScheme scheme = ColorScheme.highContrastLight();
expect(scheme.brightness, Brightness.light);
expect(scheme.primary, const Color(0xff0000ba));
expect(scheme.onPrimary, const Color(0xffffffff));
expect(scheme.primaryContainer, scheme.primary);
expect(scheme.onPrimaryContainer, scheme.onPrimary);
expect(scheme.primaryFixed, scheme.primary);
expect(scheme.primaryFixedDim, scheme.primary);
expect(scheme.onPrimaryFixed, scheme.onPrimary);
expect(scheme.onPrimaryFixedVariant, scheme.onPrimary);
expect(scheme.secondary, const Color(0xff66fff9));
expect(scheme.onSecondary, const Color(0xff000000));
expect(scheme.secondaryContainer, scheme.secondary);
expect(scheme.onSecondaryContainer, scheme.onSecondary);
expect(scheme.secondaryFixed, scheme.secondary);
expect(scheme.secondaryFixedDim, scheme.secondary);
expect(scheme.onSecondaryFixed, scheme.onSecondary);
expect(scheme.onSecondaryFixedVariant, scheme.onSecondary);
expect(scheme.tertiary, scheme.secondary);
expect(scheme.onTertiary, scheme.onSecondary);
expect(scheme.tertiaryContainer, scheme.tertiary);
expect(scheme.onTertiaryContainer, scheme.onTertiary);
expect(scheme.tertiaryFixed, scheme.tertiary);
expect(scheme.tertiaryFixedDim, scheme.tertiary);
expect(scheme.onTertiaryFixed, scheme.onTertiary);
expect(scheme.onTertiaryFixedVariant, scheme.onTertiary);
expect(scheme.error, const Color(0xff790000));
expect(scheme.onError, const Color(0xffffffff));
expect(scheme.errorContainer, scheme.error);
expect(scheme.onErrorContainer, scheme.onError);
expect(scheme.background, const Color(0xffffffff));
expect(scheme.onBackground, const Color(0xff000000));
expect(scheme.surface, const Color(0xffffffff));
expect(scheme.surfaceBright, scheme.surface);
expect(scheme.surfaceDim, scheme.surface);
expect(scheme.surfaceContainerLowest, scheme.surface);
expect(scheme.surfaceContainerLow, scheme.surface);
expect(scheme.surfaceContainer, scheme.surface);
expect(scheme.surfaceContainerHigh, scheme.surface);
expect(scheme.surfaceContainerHighest, scheme.surface);
expect(scheme.onSurface, const Color(0xff000000));
expect(scheme.surfaceVariant, scheme.surface);
expect(scheme.onSurfaceVariant, scheme.onSurface);
expect(scheme.outline, scheme.onBackground);
expect(scheme.outlineVariant, scheme.onBackground);
expect(scheme.shadow, const Color(0xff000000));
expect(scheme.scrim, const Color(0xff000000));
expect(scheme.inverseSurface, scheme.onSurface);
expect(scheme.onInverseSurface, scheme.surface);
expect(scheme.inversePrimary, scheme.onPrimary);
expect(scheme.surfaceTint, scheme.primary);
});
test('high contrast dark scheme matches the spec', () {
// Colors are based off of the Material Design baseline dark theme:
// https://material.io/design/color/dark-theme.html#ui-application
// with the new Material 3 colors defaulting to values from the M2
// baseline.
const ColorScheme scheme = ColorScheme.highContrastDark();
expect(scheme.brightness, Brightness.dark);
expect(scheme.primary, const Color(0xffefb7ff));
expect(scheme.onPrimary, const Color(0xff000000));
expect(scheme.primaryContainer, scheme.primary);
expect(scheme.onPrimaryContainer, scheme.onPrimary);
expect(scheme.primaryFixed, scheme.primary);
expect(scheme.primaryFixedDim, scheme.primary);
expect(scheme.onPrimaryFixed, scheme.onPrimary);
expect(scheme.onPrimaryFixedVariant, scheme.onPrimary);
expect(scheme.secondary, const Color(0xff66fff9));
expect(scheme.onSecondary, const Color(0xff000000));
expect(scheme.secondaryContainer, scheme.secondary);
expect(scheme.onSecondaryContainer, scheme.onSecondary);
expect(scheme.secondaryFixed, scheme.secondary);
expect(scheme.secondaryFixedDim, scheme.secondary);
expect(scheme.onSecondaryFixed, scheme.onSecondary);
expect(scheme.onSecondaryFixedVariant, scheme.onSecondary);
expect(scheme.tertiary, scheme.secondary);
expect(scheme.onTertiary, scheme.onSecondary);
expect(scheme.tertiaryContainer, scheme.tertiary);
expect(scheme.onTertiaryContainer, scheme.onTertiary);
expect(scheme.tertiaryFixed, scheme.tertiary);
expect(scheme.tertiaryFixedDim, scheme.tertiary);
expect(scheme.onTertiaryFixed, scheme.onTertiary);
expect(scheme.onTertiaryFixedVariant, scheme.onTertiary);
expect(scheme.error, const Color(0xff9b374d));
expect(scheme.onError, const Color(0xff000000));
expect(scheme.errorContainer, scheme.error);
expect(scheme.onErrorContainer, scheme.onError);
expect(scheme.background, const Color(0xff121212));
expect(scheme.onBackground, const Color(0xffffffff));
expect(scheme.surface, const Color(0xff121212));
expect(scheme.surfaceBright, scheme.surface);
expect(scheme.surfaceDim, scheme.surface);
expect(scheme.surfaceContainerLowest, scheme.surface);
expect(scheme.surfaceContainerLow, scheme.surface);
expect(scheme.surfaceContainer, scheme.surface);
expect(scheme.surfaceContainerHigh, scheme.surface);
expect(scheme.surfaceContainerHighest, scheme.surface);
expect(scheme.onSurface, const Color(0xffffffff));
expect(scheme.surfaceVariant, scheme.surface);
expect(scheme.onSurfaceVariant, scheme.onSurface);
expect(scheme.outline, scheme.onBackground);
expect(scheme.outlineVariant, scheme.onBackground);
expect(scheme.shadow, const Color(0xff000000));
expect(scheme.scrim, const Color(0xff000000));
expect(scheme.inverseSurface, scheme.onSurface);
expect(scheme.onInverseSurface, scheme.surface);
expect(scheme.inversePrimary, scheme.onPrimary);
expect(scheme.surfaceTint, scheme.primary);
});
test('can generate a light scheme from a seed color', () {
final ColorScheme scheme = ColorScheme.fromSeed(seedColor: Colors.blue);
expect(scheme.primary, const Color(0xff36618e));
expect(scheme.onPrimary, const Color(0xffffffff));
expect(scheme.primaryContainer, const Color(0xffd1e4ff));
expect(scheme.onPrimaryContainer, const Color(0xff001d36));
expect(scheme.primaryFixed, const Color(0xffd1e4ff));
expect(scheme.primaryFixedDim, const Color(0xffa0cafd));
expect(scheme.onPrimaryFixed, const Color(0xff001d36));
expect(scheme.onPrimaryFixedVariant, const Color(0xff194975));
expect(scheme.secondary, const Color(0xff535f70));
expect(scheme.onSecondary, const Color(0xffffffff));
expect(scheme.secondaryContainer, const Color(0xffd7e3f7));
expect(scheme.onSecondaryContainer, const Color(0xff101c2b));
expect(scheme.secondaryFixed, const Color(0xffd7e3f7));
expect(scheme.secondaryFixedDim, const Color(0xffbbc7db));
expect(scheme.onSecondaryFixed, const Color(0xff101c2b));
expect(scheme.onSecondaryFixedVariant, const Color(0xff3b4858));
expect(scheme.tertiary, const Color(0xff6b5778));
expect(scheme.onTertiary, const Color(0xffffffff));
expect(scheme.tertiaryContainer, const Color(0xfff2daff));
expect(scheme.onTertiaryContainer, const Color(0xff251431));
expect(scheme.tertiaryFixed, const Color(0xfff2daff));
expect(scheme.tertiaryFixedDim, const Color(0xffd6bee4));
expect(scheme.onTertiaryFixed, const Color(0xff251431));
expect(scheme.onTertiaryFixedVariant, const Color(0xff523f5f));
expect(scheme.error, const Color(0xffba1a1a));
expect(scheme.onError, const Color(0xffffffff));
expect(scheme.errorContainer, const Color(0xffffdad6));
expect(scheme.onErrorContainer, const Color(0xff410002));
expect(scheme.outline, const Color(0xff73777f));
expect(scheme.outlineVariant, const Color(0xffc3c7cf));
expect(scheme.background, const Color(0xfff8f9ff));
expect(scheme.onBackground, const Color(0xff191c20));
expect(scheme.surface, const Color(0xfff8f9ff));
expect(scheme.surfaceBright, const Color(0xfff8f9ff));
expect(scheme.surfaceDim, const Color(0xffd8dae0));
expect(scheme.surfaceContainerLowest, const Color(0xffffffff));
expect(scheme.surfaceContainerLow, const Color(0xfff2f3fa));
expect(scheme.surfaceContainer, const Color(0xffeceef4));
expect(scheme.surfaceContainerHigh, const Color(0xffe6e8ee));
expect(scheme.surfaceContainerHighest, const Color(0xffe1e2e8));
expect(scheme.onSurface, const Color(0xff191c20));
expect(scheme.surfaceVariant, const Color(0xffdfe2eb));
expect(scheme.onSurfaceVariant, const Color(0xff43474e));
expect(scheme.inverseSurface, const Color(0xff2e3135));
expect(scheme.onInverseSurface, const Color(0xffeff0f7));
expect(scheme.inversePrimary, const Color(0xffa0cafd));
expect(scheme.shadow, const Color(0xff000000));
expect(scheme.scrim, const Color(0xff000000));
expect(scheme.surfaceTint, const Color(0xff36618e));
expect(scheme.brightness, Brightness.light);
});
test('copyWith overrides given colors', () {
final ColorScheme scheme = const ColorScheme.light().copyWith(
brightness: Brightness.dark,
primary: const Color(0x00000001),
onPrimary: const Color(0x00000002),
primaryContainer: const Color(0x00000003),
onPrimaryContainer: const Color(0x00000004),
primaryFixed: const Color(0x0000001D),
primaryFixedDim: const Color(0x0000001E),
onPrimaryFixed: const Color(0x0000001F),
onPrimaryFixedVariant: const Color(0x00000020),
secondary: const Color(0x00000005),
onSecondary: const Color(0x00000006),
secondaryContainer: const Color(0x00000007),
onSecondaryContainer: const Color(0x00000008),
secondaryFixed: const Color(0x00000021),
secondaryFixedDim: const Color(0x00000022),
onSecondaryFixed: const Color(0x00000023),
onSecondaryFixedVariant: const Color(0x00000024),
tertiary: const Color(0x00000009),
onTertiary: const Color(0x0000000A),
tertiaryContainer: const Color(0x0000000B),
onTertiaryContainer: const Color(0x0000000C),
tertiaryFixed: const Color(0x00000025),
tertiaryFixedDim: const Color(0x00000026),
onTertiaryFixed: const Color(0x00000027),
onTertiaryFixedVariant: const Color(0x00000028),
error: const Color(0x0000000D),
onError: const Color(0x0000000E),
errorContainer: const Color(0x0000000F),
onErrorContainer: const Color(0x00000010),
background: const Color(0x00000011),
onBackground: const Color(0x00000012),
surface: const Color(0x00000013),
surfaceDim: const Color(0x00000029),
surfaceBright: const Color(0x0000002A),
surfaceContainerLowest: const Color(0x0000002B),
surfaceContainerLow: const Color(0x0000002C),
surfaceContainer: const Color(0x0000002D),
surfaceContainerHigh: const Color(0x0000002E),
surfaceContainerHighest: const Color(0x0000002F),
onSurface: const Color(0x00000014),
surfaceVariant: const Color(0x00000015),
onSurfaceVariant: const Color(0x00000016),
outline: const Color(0x00000017),
outlineVariant: const Color(0x00000117),
shadow: const Color(0x00000018),
scrim: const Color(0x00000118),
inverseSurface: const Color(0x00000019),
onInverseSurface: const Color(0x0000001A),
inversePrimary: const Color(0x0000001B),
surfaceTint: const Color(0x0000001C),
);
expect(scheme.brightness, Brightness.dark);
expect(scheme.primary, const Color(0x00000001));
expect(scheme.onPrimary, const Color(0x00000002));
expect(scheme.primaryContainer, const Color(0x00000003));
expect(scheme.onPrimaryContainer, const Color(0x00000004));
expect(scheme.primaryFixed, const Color(0x0000001D));
expect(scheme.primaryFixedDim, const Color(0x0000001E));
expect(scheme.onPrimaryFixed, const Color(0x0000001F));
expect(scheme.onPrimaryFixedVariant, const Color(0x00000020));
expect(scheme.secondary, const Color(0x00000005));
expect(scheme.onSecondary, const Color(0x00000006));
expect(scheme.secondaryContainer, const Color(0x00000007));
expect(scheme.onSecondaryContainer, const Color(0x00000008));
expect(scheme.secondaryFixed, const Color(0x00000021));
expect(scheme.secondaryFixedDim, const Color(0x00000022));
expect(scheme.onSecondaryFixed, const Color(0x00000023));
expect(scheme.onSecondaryFixedVariant, const Color(0x00000024));
expect(scheme.tertiary, const Color(0x00000009));
expect(scheme.onTertiary, const Color(0x0000000A));
expect(scheme.tertiaryContainer, const Color(0x0000000B));
expect(scheme.onTertiaryContainer, const Color(0x0000000C));
expect(scheme.tertiaryFixed, const Color(0x00000025));
expect(scheme.tertiaryFixedDim, const Color(0x00000026));
expect(scheme.onTertiaryFixed, const Color(0x00000027));
expect(scheme.onTertiaryFixedVariant, const Color(0x00000028));
expect(scheme.error, const Color(0x0000000D));
expect(scheme.onError, const Color(0x0000000E));
expect(scheme.errorContainer, const Color(0x0000000F));
expect(scheme.onErrorContainer, const Color(0x00000010));
expect(scheme.background, const Color(0x00000011));
expect(scheme.onBackground, const Color(0x00000012));
expect(scheme.surface, const Color(0x00000013));
expect(scheme.surfaceDim, const Color(0x00000029));
expect(scheme.surfaceBright, const Color(0x0000002A));
expect(scheme.surfaceContainerLowest, const Color(0x0000002B));
expect(scheme.surfaceContainerLow, const Color(0x0000002C));
expect(scheme.surfaceContainer, const Color(0x0000002D));
expect(scheme.surfaceContainerHigh, const Color(0x0000002E));
expect(scheme.surfaceContainerHighest, const Color(0x0000002F));
expect(scheme.onSurface, const Color(0x00000014));
expect(scheme.surfaceVariant, const Color(0x00000015));
expect(scheme.onSurfaceVariant, const Color(0x00000016));
expect(scheme.outline, const Color(0x00000017));
expect(scheme.outlineVariant, const Color(0x00000117));
expect(scheme.shadow, const Color(0x00000018));
expect(scheme.scrim, const Color(0x00000118));
expect(scheme.inverseSurface, const Color(0x00000019));
expect(scheme.onInverseSurface, const Color(0x0000001A));
expect(scheme.inversePrimary, const Color(0x0000001B));
expect(scheme.surfaceTint, const Color(0x0000001C));
});
test('can generate a dark scheme from a seed color', () {
final ColorScheme scheme = ColorScheme.fromSeed(seedColor: Colors.blue, brightness: Brightness.dark);
expect(scheme.primary, const Color(0xffa0cafd));
expect(scheme.onPrimary, const Color(0xff003258));
expect(scheme.primaryContainer, const Color(0xff194975));
expect(scheme.onPrimaryContainer, const Color(0xffd1e4ff));
expect(scheme.primaryFixed, const Color(0xffd1e4ff));
expect(scheme.primaryFixedDim, const Color(0xffa0cafd));
expect(scheme.onPrimaryFixed, const Color(0xff001d36));
expect(scheme.onPrimaryFixedVariant, const Color(0xff194975));
expect(scheme.secondary, const Color(0xffbbc7db));
expect(scheme.onSecondary, const Color(0xff253140));
expect(scheme.secondaryContainer, const Color(0xff3b4858));
expect(scheme.onSecondaryContainer, const Color(0xffd7e3f7));
expect(scheme.secondaryFixed, const Color(0xffd7e3f7));
expect(scheme.secondaryFixedDim, const Color(0xffbbc7db));
expect(scheme.onSecondaryFixed, const Color(0xff101c2b));
expect(scheme.onSecondaryFixedVariant, const Color(0xff3b4858));
expect(scheme.tertiary, const Color(0xffd6bee4));
expect(scheme.onTertiary, const Color(0xff3b2948));
expect(scheme.tertiaryContainer, const Color(0xff523f5f));
expect(scheme.onTertiaryContainer, const Color(0xfff2daff));
expect(scheme.tertiaryFixed, const Color(0xfff2daff));
expect(scheme.tertiaryFixedDim, const Color(0xffd6bee4));
expect(scheme.onTertiaryFixed, const Color(0xff251431));
expect(scheme.onTertiaryFixedVariant, const Color(0xff523f5f));
expect(scheme.error, const Color(0xffffb4ab));
expect(scheme.onError, const Color(0xff690005));
expect(scheme.errorContainer, const Color(0xff93000a));
expect(scheme.onErrorContainer, const Color(0xffffdad6));
expect(scheme.outline, const Color(0xff8d9199));
expect(scheme.outlineVariant, const Color(0xff43474e));
expect(scheme.background, const Color(0xff111418));
expect(scheme.onBackground, const Color(0xffe1e2e8));
expect(scheme.surface, const Color(0xff111418));
expect(scheme.surfaceDim, const Color(0xff111418));
expect(scheme.surfaceBright, const Color(0xff36393e));
expect(scheme.surfaceContainerLowest, const Color(0xff0b0e13));
expect(scheme.surfaceContainerLow, const Color(0xff191c20));
expect(scheme.surfaceContainer, const Color(0xff1d2024));
expect(scheme.surfaceContainerHigh, const Color(0xff272a2f));
expect(scheme.surfaceContainerHighest, const Color(0xff32353a));
expect(scheme.onSurface, const Color(0xffe1e2e8));
expect(scheme.surfaceVariant, const Color(0xff43474e));
expect(scheme.onSurfaceVariant, const Color(0xffc3c7cf));
expect(scheme.inverseSurface, const Color(0xffe1e2e8));
expect(scheme.onInverseSurface, const Color(0xff2e3135));
expect(scheme.inversePrimary, const Color(0xff36618e));
expect(scheme.shadow, const Color(0xff000000));
expect(scheme.scrim, const Color(0xff000000));
expect(scheme.surfaceTint, const Color(0xffa0cafd));
expect(scheme.brightness, Brightness.dark);
});
test('can override specific colors in a generated scheme', () {
final ColorScheme baseScheme = ColorScheme.fromSeed(seedColor: Colors.blue);
const Color primaryOverride = Color(0xffabcdef);
final ColorScheme scheme = ColorScheme.fromSeed(
seedColor: Colors.blue,
primary: primaryOverride,
);
expect(scheme.primary, primaryOverride);
// The rest should be the same.
expect(scheme.onPrimary, baseScheme.onPrimary);
expect(scheme.primaryContainer, baseScheme.primaryContainer);
expect(scheme.onPrimaryContainer, baseScheme.onPrimaryContainer);
expect(scheme.primaryFixed, baseScheme.primaryFixed);
expect(scheme.primaryFixedDim, baseScheme.primaryFixedDim);
expect(scheme.onPrimaryFixed, baseScheme.onPrimaryFixed);
expect(scheme.onPrimaryFixedVariant, baseScheme.onPrimaryFixedVariant);
expect(scheme.secondary, baseScheme.secondary);
expect(scheme.onSecondary, baseScheme.onSecondary);
expect(scheme.secondaryContainer, baseScheme.secondaryContainer);
expect(scheme.onSecondaryContainer, baseScheme.onSecondaryContainer);
expect(scheme.secondaryFixed, baseScheme.secondaryFixed);
expect(scheme.secondaryFixedDim, baseScheme.secondaryFixedDim);
expect(scheme.onSecondaryFixed, baseScheme.onSecondaryFixed);
expect(scheme.onSecondaryFixedVariant, baseScheme.onSecondaryFixedVariant);
expect(scheme.tertiary, baseScheme.tertiary);
expect(scheme.onTertiary, baseScheme.onTertiary);
expect(scheme.tertiaryContainer, baseScheme.tertiaryContainer);
expect(scheme.onTertiaryContainer, baseScheme.onTertiaryContainer);
expect(scheme.tertiaryFixed, baseScheme.tertiaryFixed);
expect(scheme.tertiaryFixedDim, baseScheme.tertiaryFixedDim);
expect(scheme.onTertiaryFixed, baseScheme.onTertiaryFixed);
expect(scheme.onTertiaryFixedVariant, baseScheme.onTertiaryFixedVariant);
expect(scheme.error, baseScheme.error);
expect(scheme.onError, baseScheme.onError);
expect(scheme.errorContainer, baseScheme.errorContainer);
expect(scheme.onErrorContainer, baseScheme.onErrorContainer);
expect(scheme.outline, baseScheme.outline);
expect(scheme.outlineVariant, baseScheme.outlineVariant);
expect(scheme.background, baseScheme.background);
expect(scheme.onBackground, baseScheme.onBackground);
expect(scheme.surface, baseScheme.surface);
expect(scheme.surfaceBright, baseScheme.surfaceBright);
expect(scheme.surfaceDim, baseScheme.surfaceDim);
expect(scheme.surfaceContainerLowest, baseScheme.surfaceContainerLowest);
expect(scheme.surfaceContainerLow, baseScheme.surfaceContainerLow);
expect(scheme.surfaceContainer, baseScheme.surfaceContainer);
expect(scheme.surfaceContainerHigh, baseScheme.surfaceContainerHigh);
expect(scheme.surfaceContainerHighest, baseScheme.surfaceContainerHighest);
expect(scheme.onSurface, baseScheme.onSurface);
expect(scheme.surfaceVariant, baseScheme.surfaceVariant);
expect(scheme.onSurfaceVariant, baseScheme.onSurfaceVariant);
expect(scheme.inverseSurface, baseScheme.inverseSurface);
expect(scheme.onInverseSurface, baseScheme.onInverseSurface);
expect(scheme.inversePrimary, baseScheme.inversePrimary);
expect(scheme.shadow, baseScheme.shadow);
expect(scheme.scrim, baseScheme.shadow);
expect(scheme.surfaceTint, baseScheme.surfaceTint);
expect(scheme.brightness, baseScheme.brightness);
});
test('can generate a light scheme from an imageProvider', () async {
final Uint8List blueSquareBytes = Uint8List.fromList(kBlueSquarePng);
final ImageProvider image = MemoryImage(blueSquareBytes);
final ColorScheme scheme =
await ColorScheme.fromImageProvider(provider: image);
expect(scheme.brightness, Brightness.light);
expect(scheme.primary, const Color(0xff575992));
expect(scheme.onPrimary, const Color(0xffffffff));
expect(scheme.primaryContainer, const Color(0xffe1e0ff));
expect(scheme.onPrimaryContainer, const Color(0xff13144b));
expect(scheme.primaryFixed, const Color(0xffe1e0ff));
expect(scheme.primaryFixedDim, const Color(0xffc0c1ff));
expect(scheme.onPrimaryFixed, const Color(0xff13144b));
expect(scheme.onPrimaryFixedVariant, const Color(0xff3f4178));
expect(scheme.secondary, const Color(0xff5d5c72));
expect(scheme.onSecondary, const Color(0xffffffff));
expect(scheme.secondaryContainer, const Color(0xffe2e0f9));
expect(scheme.onSecondaryContainer, const Color(0xff191a2c));
expect(scheme.secondaryFixed, const Color(0xffe2e0f9));
expect(scheme.secondaryFixedDim, const Color(0xffc6c4dd));
expect(scheme.onSecondaryFixed, const Color(0xff191a2c));
expect(scheme.onSecondaryFixedVariant, const Color(0xff454559));
expect(scheme.tertiary, const Color(0xff79536a));
expect(scheme.onTertiary, const Color(0xffffffff));
expect(scheme.tertiaryContainer, const Color(0xffffd8ec));
expect(scheme.onTertiaryContainer, const Color(0xff2e1125));
expect(scheme.tertiaryFixed, const Color(0xffffd8ec));
expect(scheme.tertiaryFixedDim, const Color(0xffe9b9d3));
expect(scheme.onTertiaryFixed, const Color(0xff2e1125));
expect(scheme.onTertiaryFixedVariant, const Color(0xff5f3c51));
expect(scheme.error, const Color(0xffba1a1a));
expect(scheme.onError, const Color(0xffffffff));
expect(scheme.errorContainer, const Color(0xffffdad6));
expect(scheme.onErrorContainer, const Color(0xff410002));
expect(scheme.background, const Color(0xfffcf8ff));
expect(scheme.onBackground, const Color(0xff1b1b21));
expect(scheme.surface, const Color(0xfffcf8ff));
expect(scheme.surfaceDim, const Color(0xffdcd9e0));
expect(scheme.surfaceBright, const Color(0xfffcf8ff));
expect(scheme.surfaceContainerLowest, const Color(0xffffffff));
expect(scheme.surfaceContainerLow, const Color(0xfff6f2fa));
expect(scheme.surfaceContainer, const Color(0xfff0ecf4));
expect(scheme.surfaceContainerHigh, const Color(0xffeae7ef));
expect(scheme.surfaceContainerHighest, const Color(0xffe4e1e9));
expect(scheme.onSurface, const Color(0xff1b1b21));
expect(scheme.surfaceVariant, const Color(0xffe4e1ec));
expect(scheme.onSurfaceVariant, const Color(0xff46464f));
expect(scheme.outline, const Color(0xff777680));
expect(scheme.outlineVariant, const Color(0xffc8c5d0));
expect(scheme.shadow, const Color(0xff000000));
expect(scheme.scrim, const Color(0xff000000));
expect(scheme.inverseSurface, const Color(0xff303036));
expect(scheme.onInverseSurface, const Color(0xfff3eff7));
expect(scheme.inversePrimary, const Color(0xffc0c1ff));
expect(scheme.surfaceTint, const Color(0xff575992));
}, skip: isBrowser, // [intended] uses dart:typed_data.
);
test('can generate a dark scheme from an imageProvider', () async {
final Uint8List blueSquareBytes = Uint8List.fromList(kBlueSquarePng);
final ImageProvider image = MemoryImage(blueSquareBytes);
final ColorScheme scheme = await ColorScheme.fromImageProvider(
provider: image, brightness: Brightness.dark);
expect(scheme.primary, const Color(0xffc0c1ff));
expect(scheme.onPrimary, const Color(0xff292a60));
expect(scheme.primaryContainer, const Color(0xff3f4178));
expect(scheme.onPrimaryContainer, const Color(0xffe1e0ff));
expect(scheme.primaryFixed, const Color(0xffe1e0ff));
expect(scheme.primaryFixedDim, const Color(0xffc0c1ff));
expect(scheme.onPrimaryFixed, const Color(0xff13144b));
expect(scheme.onPrimaryFixedVariant, const Color(0xff3f4178));
expect(scheme.secondary, const Color(0xffc6c4dd));
expect(scheme.onSecondary, const Color(0xff2e2f42));
expect(scheme.secondaryContainer, const Color(0xff454559));
expect(scheme.onSecondaryContainer, const Color(0xffe2e0f9));
expect(scheme.secondaryFixed, const Color(0xffe2e0f9));
expect(scheme.secondaryFixedDim, const Color(0xffc6c4dd));
expect(scheme.onSecondaryFixed, const Color(0xff191a2c));
expect(scheme.onSecondaryFixedVariant, const Color(0xff454559));
expect(scheme.tertiary, const Color(0xffe9b9d3));
expect(scheme.onTertiary, const Color(0xff46263a));
expect(scheme.tertiaryContainer, const Color(0xff5f3c51));
expect(scheme.onTertiaryContainer, const Color(0xffffd8ec));
expect(scheme.tertiaryFixed, const Color(0xffffd8ec));
expect(scheme.tertiaryFixedDim, const Color(0xffe9b9d3));
expect(scheme.onTertiaryFixed, const Color(0xff2e1125));
expect(scheme.onTertiaryFixedVariant, const Color(0xff5f3c51));
expect(scheme.error, const Color(0xffffb4ab));
expect(scheme.onError, const Color(0xff690005));
expect(scheme.errorContainer, const Color(0xff93000a));
expect(scheme.onErrorContainer, const Color(0xffffdad6));
expect(scheme.background, const Color(0xff131318));
expect(scheme.onBackground, const Color(0xffe4e1e9));
expect(scheme.surface, const Color(0xff131318));
expect(scheme.surfaceDim, const Color(0xff131318));
expect(scheme.surfaceBright, const Color(0xff39383f));
expect(scheme.surfaceContainerLowest, const Color(0xff0e0e13));
expect(scheme.surfaceContainerLow, const Color(0xff1b1b21));
expect(scheme.surfaceContainer, const Color(0xff1f1f25));
expect(scheme.surfaceContainerHigh, const Color(0xff2a292f));
expect(scheme.surfaceContainerHighest, const Color(0xff35343a));
expect(scheme.onSurface, const Color(0xffe4e1e9));
expect(scheme.surfaceVariant, const Color(0xff46464f));
expect(scheme.onSurfaceVariant, const Color(0xffc8c5d0));
expect(scheme.outline, const Color(0xff918f9a));
expect(scheme.outlineVariant, const Color(0xff46464f));
expect(scheme.inverseSurface, const Color(0xffe4e1e9));
expect(scheme.onInverseSurface, const Color(0xff303036));
expect(scheme.inversePrimary, const Color(0xff575992));
expect(scheme.surfaceTint, const Color(0xffc0c1ff));
}, skip: isBrowser, // [intended] uses dart:isolate and io.
);
test('fromImageProvider() propagates TimeoutException when image cannot be rendered', () async {
final Uint8List blueSquareBytes = Uint8List.fromList(kBlueSquarePng);
// Corrupt the image's bytelist so it cannot be read.
final Uint8List corruptImage = blueSquareBytes.sublist(5);
final ImageProvider image = MemoryImage(corruptImage);
expect(() async => ColorScheme.fromImageProvider(provider: image), throwsA(
isA<Exception>().having((Exception e) => e.toString(),
'Timeout occurred trying to load image', contains('TimeoutException')),
),
);
});
testWidgets('generated scheme "on" colors meet a11y contrast guidelines', (WidgetTester tester) async {
final ColorScheme colors = ColorScheme.fromSeed(seedColor: Colors.teal);
Widget label(String text, Color textColor, Color background) {
return Container(
color: background,
padding: const EdgeInsets.all(8),
child: Text(text, style: TextStyle(color: textColor)),
);
}
await tester.pumpWidget(
MaterialApp(
theme: ThemeData.from(colorScheme: colors),
home: Scaffold(
body: Column(
children: <Widget>[
label('primary', colors.onPrimary, colors.primary),
label('secondary', colors.onSecondary, colors.secondary),
label('tertiary', colors.onTertiary, colors.tertiary),
label('error', colors.onError, colors.error),
label('background', colors.onBackground, colors.background),
label('surface', colors.onSurface, colors.surface),
],
),
),
),
);
await expectLater(tester, meetsGuideline(textContrastGuideline));
},
skip: isBrowser, // https://github.com/flutter/flutter/issues/44115
);
}
| flutter/packages/flutter/test/material/color_scheme_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/color_scheme_test.dart",
"repo_id": "flutter",
"token_count": 13023
} | 689 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/semantics_tester.dart';
void main() {
testWidgets('Material2 - Drawer control test', (WidgetTester tester) async {
const Key containerKey = Key('container');
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Scaffold(
drawer: Drawer(
child: ListView(
children: <Widget>[
DrawerHeader(
child: Container(
key: containerKey,
child: const Text('header'),
),
),
const ListTile(
leading: Icon(Icons.archive),
title: Text('Archive'),
),
],
),
),
),
),
);
expect(find.text('Archive'), findsNothing);
final ScaffoldState state = tester.firstState(find.byType(Scaffold));
state.openDrawer();
await tester.pump();
await tester.pump(const Duration(seconds: 1));
expect(find.text('Archive'), findsOneWidget);
RenderBox box = tester.renderObject(find.byType(DrawerHeader));
expect(box.size.height, equals(160.0 + 8.0 + 1.0)); // height + bottom margin + bottom edge
final double drawerWidth = box.size.width;
final double drawerHeight = box.size.height;
box = tester.renderObject(find.byKey(containerKey));
expect(box.size.width, equals(drawerWidth - 2 * 16.0));
expect(box.size.height, equals(drawerHeight - 2 * 16.0));
expect(find.text('header'), findsOneWidget);
});
testWidgets('Material3 - Drawer control test', (WidgetTester tester) async {
const Key containerKey = Key('container');
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: true),
home: Scaffold(
drawer: Drawer(
child: ListView(
children: <Widget>[
DrawerHeader(
child: Container(
key: containerKey,
child: const Text('header'),
),
),
const ListTile(
leading: Icon(Icons.archive),
title: Text('Archive'),
),
],
),
),
),
),
);
expect(find.text('Archive'), findsNothing);
final ScaffoldState state = tester.firstState(find.byType(Scaffold));
state.openDrawer();
await tester.pump();
await tester.pump(const Duration(seconds: 1));
expect(find.text('Archive'), findsOneWidget);
RenderBox box = tester.renderObject(find.byType(DrawerHeader));
expect(box.size.height, equals(160.0 + 8.0 + 1.0)); // height + bottom margin + bottom edge
final double drawerWidth = box.size.width;
final double drawerHeight = box.size.height;
box = tester.renderObject(find.byKey(containerKey));
expect(box.size.width, equals(drawerWidth - 2 * 16.0));
expect(box.size.height, equals(drawerHeight - 2 * 16.0 - 1.0)); // Header divider thickness is 1.0 in Material 3.
expect(find.text('header'), findsOneWidget);
});
testWidgets('Drawer dismiss barrier has label', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
drawer: Drawer(),
),
),
);
final ScaffoldState state = tester.firstState(find.byType(Scaffold));
state.openDrawer();
await tester.pump();
await tester.pump(const Duration(seconds: 1));
expect(semantics, includesNodeWith(
label: const DefaultMaterialLocalizations().modalBarrierDismissLabel,
actions: <SemanticsAction>[SemanticsAction.tap],
));
semantics.dispose();
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
testWidgets('Drawer dismiss barrier has no label', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
drawer: Drawer(),
),
),
);
final ScaffoldState state = tester.firstState(find.byType(Scaffold));
state.openDrawer();
await tester.pump();
await tester.pump(const Duration(seconds: 1));
expect(semantics, isNot(includesNodeWith(
label: const DefaultMaterialLocalizations().modalBarrierDismissLabel,
actions: <SemanticsAction>[SemanticsAction.tap],
)));
semantics.dispose();
}, variant: TargetPlatformVariant.only(TargetPlatform.android));
testWidgets('Scaffold drawerScrimColor', (WidgetTester tester) async {
// The scrim is a Container within a Semantics node labeled "Dismiss",
// within a DrawerController. Sorry.
Container getScrim() {
return tester.widget<Container>(
find.descendant(
of: find.descendant(
of: find.byType(DrawerController),
matching: find.byWidgetPredicate((Widget widget) {
return widget is Semantics
&& widget.properties.label == 'Dismiss';
}),
),
matching: find.byType(Container),
),
);
}
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
Widget buildFrame({ Color? drawerScrimColor }) {
return MaterialApp(
home: Scaffold(
key: scaffoldKey,
drawerScrimColor: drawerScrimColor,
drawer: Drawer(
child: Builder(
builder: (BuildContext context) {
return GestureDetector(
onTap: () { Navigator.pop(context); }, // close drawer
);
},
),
),
),
);
}
// Default drawerScrimColor
await tester.pumpWidget(buildFrame());
scaffoldKey.currentState!.openDrawer();
await tester.pumpAndSettle();
expect(getScrim().color, Colors.black54);
await tester.tap(find.byType(Drawer));
await tester.pumpAndSettle();
expect(find.byType(Drawer), findsNothing);
// Specific drawerScrimColor
await tester.pumpWidget(buildFrame(drawerScrimColor: const Color(0xFF323232)));
scaffoldKey.currentState!.openDrawer();
await tester.pumpAndSettle();
expect(getScrim().color, const Color(0xFF323232));
await tester.tap(find.byType(Drawer));
await tester.pumpAndSettle();
expect(find.byType(Drawer), findsNothing);
});
testWidgets('Open/close drawers by flinging', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
drawer: Drawer(
child: Text('start drawer'),
),
endDrawer: Drawer(
child: Text('end drawer'),
),
),
),
);
// In the beginning, drawers are closed
final ScaffoldState state = tester.firstState(find.byType(Scaffold));
expect(state.isDrawerOpen, equals(false));
expect(state.isEndDrawerOpen, equals(false));
final Size size = tester.getSize(find.byType(Scaffold));
// A fling from the left opens the start drawer
await tester.flingFrom(Offset(0, size.height / 2), const Offset(80, 0), 500);
await tester.pumpAndSettle();
expect(state.isDrawerOpen, equals(true));
expect(state.isEndDrawerOpen, equals(false));
// Now, a fling from the right closes the drawer
await tester.flingFrom(Offset(size.width - 1, size.height / 2), const Offset(-80, 0), 500);
await tester.pumpAndSettle();
expect(state.isDrawerOpen, equals(false));
expect(state.isEndDrawerOpen, equals(false));
// Another fling from the right opens the end drawer
await tester.flingFrom(Offset(size.width - 1, size.height / 2), const Offset(-80, 0), 500);
await tester.pumpAndSettle();
expect(state.isDrawerOpen, equals(false));
expect(state.isEndDrawerOpen, equals(true));
// And a fling from the left closes it
await tester.flingFrom( Offset(0, size.height / 2), const Offset(80, 0), 500);
await tester.pumpAndSettle();
expect(state.isDrawerOpen, equals(false));
expect(state.isEndDrawerOpen, equals(false));
});
testWidgets('Scaffold.drawer - null restorationId ', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(
MaterialApp(
restorationScopeId: 'app',
home: Scaffold(
key: scaffoldKey,
drawer: const Text('drawer'),
body: Container(),
),
),
);
await tester.pump(); // no effect
expect(find.text('drawer'), findsNothing);
scaffoldKey.currentState!.openDrawer();
await tester.pumpAndSettle();
expect(find.text('drawer'), findsOneWidget);
await tester.restartAndRestore();
// Drawer state should not have been saved.
expect(find.text('drawer'), findsNothing);
});
testWidgets('Scaffold.endDrawer - null restorationId ', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(
MaterialApp(
restorationScopeId: 'app',
home: Scaffold(
key: scaffoldKey,
drawer: const Text('endDrawer'),
body: Container(),
),
),
);
await tester.pump(); // no effect
expect(find.text('endDrawer'), findsNothing);
scaffoldKey.currentState!.openDrawer();
await tester.pumpAndSettle();
expect(find.text('endDrawer'), findsOneWidget);
await tester.restartAndRestore();
// Drawer state should not have been saved.
expect(find.text('endDrawer'), findsNothing);
});
testWidgets('Scaffold.drawer state restoration test', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(
MaterialApp(
restorationScopeId: 'app',
home: Scaffold(
key: scaffoldKey,
restorationId: 'scaffold',
drawer: const Text('drawer'),
body: Container(),
),
),
);
await tester.pump(); // no effect
expect(find.text('drawer'), findsNothing);
scaffoldKey.currentState!.openDrawer();
await tester.pumpAndSettle();
expect(find.text('drawer'), findsOneWidget);
await tester.restartAndRestore();
expect(find.text('drawer'), findsOneWidget);
final TestRestorationData data = await tester.getRestorationData();
await tester.tapAt(const Offset(750.0, 100.0)); // on the mask
await tester.pumpAndSettle();
expect(find.text('drawer'), findsNothing);
await tester.restoreFrom(data);
expect(find.text('drawer'), findsOneWidget);
});
testWidgets('Scaffold.endDrawer state restoration test', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(
MaterialApp(
restorationScopeId: 'app',
home: Scaffold(
key: scaffoldKey,
restorationId: 'scaffold',
endDrawer: const Text('endDrawer'),
body: Container(),
),
),
);
await tester.pump(); // no effect
expect(find.text('endDrawer'), findsNothing);
scaffoldKey.currentState!.openEndDrawer();
await tester.pumpAndSettle();
expect(find.text('endDrawer'), findsOneWidget);
await tester.restartAndRestore();
expect(find.text('endDrawer'), findsOneWidget);
final TestRestorationData data = await tester.getRestorationData();
await tester.tapAt(const Offset(750.0, 100.0)); // on the mask
await tester.pumpAndSettle();
expect(find.text('endDrawer'), findsNothing);
await tester.restoreFrom(data);
expect(find.text('endDrawer'), findsOneWidget);
});
testWidgets('Both drawer and endDrawer state restoration test', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(
MaterialApp(
restorationScopeId: 'app',
home: Scaffold(
restorationId: 'scaffold',
key: scaffoldKey,
drawer: const Text('drawer'),
endDrawer: const Text('endDrawer'),
body: Container(),
),
),
);
await tester.pump(); // no effect
expect(find.text('drawer'), findsNothing);
expect(find.text('endDrawer'), findsNothing);
scaffoldKey.currentState!.openDrawer();
await tester.pumpAndSettle();
expect(find.text('drawer'), findsOneWidget);
expect(find.text('endDrawer'), findsNothing);
await tester.restartAndRestore();
expect(find.text('drawer'), findsOneWidget);
expect(find.text('endDrawer'), findsNothing);
TestRestorationData data = await tester.getRestorationData();
await tester.tapAt(const Offset(750.0, 100.0)); // on the mask
await tester.pumpAndSettle();
expect(find.text('drawer'), findsNothing);
expect(find.text('endDrawer'), findsNothing);
await tester.restoreFrom(data);
expect(find.text('drawer'), findsOneWidget);
expect(find.text('endDrawer'), findsNothing);
await tester.tapAt(const Offset(750.0, 100.0)); // on the mask
await tester.pumpAndSettle();
expect(find.text('drawer'), findsNothing);
expect(find.text('endDrawer'), findsNothing);
scaffoldKey.currentState!.openEndDrawer();
await tester.pumpAndSettle();
expect(find.text('drawer'), findsNothing);
expect(find.text('endDrawer'), findsOneWidget);
await tester.restartAndRestore();
expect(find.text('drawer'), findsNothing);
expect(find.text('endDrawer'), findsOneWidget);
data = await tester.getRestorationData();
await tester.tapAt(const Offset(750.0, 100.0)); // on the mask
await tester.pumpAndSettle();
expect(find.text('drawer'), findsNothing);
expect(find.text('endDrawer'), findsNothing);
await tester.restoreFrom(data);
expect(find.text('drawer'), findsNothing);
expect(find.text('endDrawer'), findsOneWidget);
});
testWidgets('ScaffoldState close drawer', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
key: scaffoldKey,
drawer: const Text('Drawer'),
body: Container(),
),
),
);
expect(find.text('Drawer'), findsNothing);
scaffoldKey.currentState!.openDrawer();
await tester.pumpAndSettle();
expect(find.text('Drawer'), findsOneWidget);
scaffoldKey.currentState!.closeDrawer();
await tester.pumpAndSettle();
expect(find.text('Drawer'), findsNothing);
});
testWidgets('ScaffoldState close drawer do not crash if drawer is already closed', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
key: scaffoldKey,
drawer: const Text('Drawer'),
body: Container(),
),
),
);
expect(find.text('Drawer'), findsNothing);
scaffoldKey.currentState!.closeDrawer();
await tester.pumpAndSettle();
expect(find.text('Drawer'), findsNothing);
});
testWidgets('Disposing drawer does not crash if drawer is open and framework is locked', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/34978
addTearDown(tester.view.reset);
tester.view.physicalSize = const Size(1800.0, 2400.0);
await tester.pumpWidget(
MaterialApp(
home: OrientationBuilder(
builder: (BuildContext context, Orientation orientation) {
switch (orientation) {
case Orientation.portrait:
return Scaffold(
drawer: const Text('drawer'),
body: Container(),
);
case Orientation.landscape:
return Scaffold(
appBar: AppBar(),
body: Container(),
);
}
},
),
),
);
expect(find.text('drawer'), findsNothing);
// Using a global key is a workaround for this issue.
final ScaffoldState portraitScaffoldState = tester.firstState(find.byType(Scaffold));
portraitScaffoldState.openDrawer();
await tester.pumpAndSettle();
expect(find.text('drawer'), findsOneWidget);
// Change the orientation and cause the drawer controller to be disposed
// while the framework is locked.
tester.view.physicalSize = const Size(2400.0, 1800.0);
await tester.pumpAndSettle();
expect(find.byType(BackButton), findsNothing);
});
testWidgets('Disposing endDrawer does not crash if endDrawer is open and framework is locked', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/34978
addTearDown(tester.view.reset);
tester.view.physicalSize = const Size(1800.0, 2400.0);
await tester.pumpWidget(
MaterialApp(
home: OrientationBuilder(
builder: (BuildContext context, Orientation orientation) {
switch (orientation) {
case Orientation.portrait:
return Scaffold(
endDrawer: const Text('endDrawer'),
body: Container(),
);
case Orientation.landscape:
return Scaffold(
appBar: AppBar(),
body: Container(),
);
}
},
),
),
);
expect(find.text('endDrawer'), findsNothing);
// Using a global key is a workaround for this issue.
final ScaffoldState portraitScaffoldState = tester.firstState(find.byType(Scaffold));
portraitScaffoldState.openEndDrawer();
await tester.pumpAndSettle();
expect(find.text('endDrawer'), findsOneWidget);
// Change the orientation and cause the drawer controller to be disposed
// while the framework is locked.
tester.view.physicalSize = const Size(2400.0, 1800.0);
await tester.pumpAndSettle();
expect(find.byType(BackButton), findsNothing);
});
testWidgets('ScaffoldState close end drawer', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
key: scaffoldKey,
endDrawer: const Text('endDrawer'),
body: Container(),
),
),
);
expect(find.text('endDrawer'), findsNothing);
scaffoldKey.currentState!.openEndDrawer();
await tester.pumpAndSettle();
expect(find.text('endDrawer'), findsOneWidget);
scaffoldKey.currentState!.closeEndDrawer();
await tester.pumpAndSettle();
expect(find.text('endDrawer'), findsNothing);
});
testWidgets('Drawer width defaults to Material spec', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
drawer: Drawer(),
),
),
);
final ScaffoldState state = tester.firstState(find.byType(Scaffold));
state.openDrawer();
await tester.pump();
await tester.pump(const Duration(seconds: 1));
final RenderBox box = tester.renderObject(find.byType(Drawer));
expect(box.size.width, equals(304.0));
});
testWidgets('Drawer width can be customized by parameter', (WidgetTester tester) async {
const double smallWidth = 200;
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
drawer: Drawer(
width: smallWidth,
),
),
),
);
final ScaffoldState state = tester.firstState(find.byType(Scaffold));
state.openDrawer();
await tester.pumpAndSettle();
final RenderBox box = tester.renderObject(find.byType(Drawer));
expect(box.size.width, equals(smallWidth));
});
testWidgets('Material3 - Drawer default shape (ltr)', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const Directionality(
textDirection: TextDirection.ltr,
child: Scaffold(
drawer: Drawer(),
endDrawer: Drawer(),
),
),
),
);
final Finder drawerMaterial = find.descendant(
of: find.byType(Drawer),
matching: find.byType(Material),
);
final ScaffoldState state = tester.firstState(find.byType(Scaffold));
// Open the drawer.
state.openDrawer();
await tester.pump();
await tester.pump(const Duration(seconds: 1));
// Test the drawer shape.
Material material = tester.widget<Material>(drawerMaterial);
expect(
material.shape,
const RoundedRectangleBorder(
borderRadius: BorderRadius.only(
topRight: Radius.circular(16.0),
bottomRight: Radius.circular(16.0),
),
),
);
// Close the opened drawer.
await tester.tapAt(const Offset(750, 300));
await tester.pumpAndSettle();
// Open the end drawer.
state.openEndDrawer();
await tester.pump();
await tester.pump(const Duration(seconds: 1));
// Test the end drawer shape.
material = tester.widget<Material>(drawerMaterial);
expect(
material.shape,
const RoundedRectangleBorder(
borderRadius: BorderRadius.only(
topLeft: Radius.circular(16.0),
bottomLeft: Radius.circular(16.0),
),
),
);
});
testWidgets('Material3 - Drawer default shape (rtl)', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const Directionality(
textDirection: TextDirection.rtl,
child: Scaffold(
drawer: Drawer(),
endDrawer: Drawer(),
),
),
),
);
final Finder drawerMaterial = find.descendant(
of: find.byType(Drawer),
matching: find.byType(Material),
);
final ScaffoldState state = tester.firstState(find.byType(Scaffold));
// Open the drawer.
state.openDrawer();
await tester.pump();
await tester.pump(const Duration(seconds: 1));
// Test the drawer shape.
Material material = tester.widget<Material>(drawerMaterial);
expect(
material.shape,
const RoundedRectangleBorder(
borderRadius: BorderRadius.only(
topLeft: Radius.circular(16.0),
bottomLeft: Radius.circular(16.0),
),
),
);
// Close the opened drawer.
await tester.tapAt(const Offset(750, 300));
await tester.pumpAndSettle();
// Open the end drawer.
state.openEndDrawer();
await tester.pump();
await tester.pump(const Duration(seconds: 1));
// Test the end drawer shape.
material = tester.widget<Material>(drawerMaterial);
expect(
material.shape,
const RoundedRectangleBorder(
borderRadius: BorderRadius.only(
topRight: Radius.circular(16.0),
bottomRight: Radius.circular(16.0),
),
),
);
});
testWidgets('Material3 - Drawer clip behavior', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const Scaffold(
drawer: Drawer(),
),
),
);
final Finder drawerMaterial = find.descendant(
of: find.byType(Drawer),
matching: find.byType(Material),
);
final ScaffoldState state = tester.firstState(find.byType(Scaffold));
// Open the drawer.
state.openDrawer();
await tester.pump();
await tester.pump(const Duration(seconds: 1));
// Test default clip behavior.
Material material = tester.widget<Material>(drawerMaterial);
expect(material.clipBehavior, Clip.hardEdge);
state.closeDrawer();
await tester.pumpAndSettle();
// Provide a custom clip behavior.
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const Scaffold(
drawer: Drawer(
clipBehavior: Clip.antiAlias,
),
),
),
);
// Open the drawer again.
state.openDrawer();
await tester.pump();
await tester.pump(const Duration(seconds: 1));
// Clip behavior is now updated.
material = tester.widget<Material>(drawerMaterial);
expect(material.clipBehavior, Clip.antiAlias);
});
group('Material 2', () {
// These tests are only relevant for Material 2. Once Material 2
// support is deprecated and the APIs are removed, these tests
// can be deleted.
testWidgets('Material2 - Drawer default shape', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: const Scaffold(
drawer: Drawer(),
endDrawer: Drawer(),
),
),
);
final Finder drawerMaterial = find.descendant(
of: find.byType(Drawer),
matching: find.byType(Material),
);
final ScaffoldState state = tester.firstState(find.byType(Scaffold));
// Open the drawer.
state.openDrawer();
await tester.pump();
await tester.pump(const Duration(seconds: 1));
// Test the drawer shape.
Material material = tester.widget<Material>(drawerMaterial);
expect(material.shape, null);
// Close the opened drawer.
await tester.tapAt(const Offset(750, 300));
await tester.pumpAndSettle();
// Open the end drawer.
state.openEndDrawer();
await tester.pump();
await tester.pump(const Duration(seconds: 1));
// Test the end drawer shape.
material = tester.widget<Material>(drawerMaterial);
expect(material.shape, null);
});
testWidgets('Material2 - Drawer clip behavior', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: const Scaffold(
drawer: Drawer(),
),
),
);
final Finder drawerMaterial = find.descendant(
of: find.byType(Drawer),
matching: find.byType(Material),
);
final ScaffoldState state = tester.firstState(find.byType(Scaffold));
// Open the drawer.
state.openDrawer();
await tester.pump();
await tester.pump(const Duration(seconds: 1));
// Test default clip behavior.
Material material = tester.widget<Material>(drawerMaterial);
expect(material.clipBehavior, Clip.none);
state.closeDrawer();
await tester.pumpAndSettle();
// Provide a shape and custom clip behavior.
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: const Scaffold(
drawer: Drawer(
clipBehavior: Clip.hardEdge,
shape: RoundedRectangleBorder(),
),
),
),
);
// Open the drawer again.
state.openDrawer();
await tester.pump();
await tester.pump(const Duration(seconds: 1));
// Clip behavior is now updated.
material = tester.widget<Material>(drawerMaterial);
expect(material.clipBehavior, Clip.hardEdge);
});
});
}
| flutter/packages/flutter/test/material/drawer_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/drawer_test.dart",
"repo_id": "flutter",
"token_count": 11364
} | 690 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('FilledButtonThemeData lerp special cases', () {
expect(FilledButtonThemeData.lerp(null, null, 0), null);
const FilledButtonThemeData data = FilledButtonThemeData();
expect(identical(FilledButtonThemeData.lerp(data, data, 0.5), data), true);
});
testWidgets('Passing no FilledButtonTheme returns defaults', (WidgetTester tester) async {
const ColorScheme colorScheme = ColorScheme.light();
await tester.pumpWidget(
MaterialApp(
theme: ThemeData.from(colorScheme: colorScheme),
home: Scaffold(
body: Center(
child: FilledButton(
onPressed: () { },
child: const Text('button'),
),
),
),
),
);
final Finder buttonMaterial = find.descendant(
of: find.byType(FilledButton),
matching: find.byType(Material),
);
final Material material = tester.widget<Material>(buttonMaterial);
expect(material.animationDuration, const Duration(milliseconds: 200));
expect(material.borderRadius, null);
expect(material.color, colorScheme.primary);
expect(material.elevation, 0);
expect(material.shadowColor, const Color(0xff000000));
expect(material.shape, const StadiumBorder());
expect(material.textStyle!.color, colorScheme.onPrimary);
expect(material.textStyle!.fontFamily, 'Roboto');
expect(material.textStyle!.fontSize, 14);
expect(material.textStyle!.fontWeight, FontWeight.w500);
final Align align = tester.firstWidget<Align>(find.ancestor(of: find.text('button'), matching: find.byType(Align)));
expect(align.alignment, Alignment.center);
});
group('[Theme, TextTheme, FilledButton style overrides]', () {
const Color foregroundColor = Color(0xff000001);
const Color backgroundColor = Color(0xff000002);
const Color disabledForegroundColor = Color(0xff000003);
const Color disabledBackgroundColor = Color(0xff000004);
const Color shadowColor = Color(0xff000005);
const double elevation = 1;
const TextStyle textStyle = TextStyle(fontSize: 12.0);
const EdgeInsets padding = EdgeInsets.all(3);
const Size minimumSize = Size(200, 200);
const BorderSide side = BorderSide(color: Colors.green, width: 2);
const OutlinedBorder shape = RoundedRectangleBorder(side: side, borderRadius: BorderRadius.all(Radius.circular(2)));
const MouseCursor enabledMouseCursor = SystemMouseCursors.text;
const MouseCursor disabledMouseCursor = SystemMouseCursors.grab;
const MaterialTapTargetSize tapTargetSize = MaterialTapTargetSize.shrinkWrap;
const Duration animationDuration = Duration(milliseconds: 25);
const bool enableFeedback = false;
const AlignmentGeometry alignment = Alignment.centerLeft;
final ButtonStyle style = FilledButton.styleFrom(
foregroundColor: foregroundColor,
backgroundColor: backgroundColor,
disabledForegroundColor: disabledForegroundColor,
disabledBackgroundColor: disabledBackgroundColor,
shadowColor: shadowColor,
elevation: elevation,
textStyle: textStyle,
padding: padding,
minimumSize: minimumSize,
side: side,
shape: shape,
enabledMouseCursor: enabledMouseCursor,
disabledMouseCursor: disabledMouseCursor,
tapTargetSize: tapTargetSize,
animationDuration: animationDuration,
enableFeedback: enableFeedback,
alignment: alignment,
);
Widget buildFrame({ ButtonStyle? buttonStyle, ButtonStyle? themeStyle, ButtonStyle? overallStyle }) {
final Widget child = Builder(
builder: (BuildContext context) {
return FilledButton(
style: buttonStyle,
onPressed: () { },
child: const Text('button'),
);
},
);
return MaterialApp(
theme: ThemeData.from(colorScheme: const ColorScheme.light()).copyWith(
filledButtonTheme: FilledButtonThemeData(style: overallStyle),
),
home: Scaffold(
body: Center(
// If the FilledButtonTheme widget is present, it's used
// instead of the Theme's ThemeData.FilledButtonTheme.
child: themeStyle == null ? child : FilledButtonTheme(
data: FilledButtonThemeData(style: themeStyle),
child: child,
),
),
),
);
}
final Finder findMaterial = find.descendant(
of: find.byType(FilledButton),
matching: find.byType(Material),
);
final Finder findInkWell = find.descendant(
of: find.byType(FilledButton),
matching: find.byType(InkWell),
);
const Set<MaterialState> enabled = <MaterialState>{};
const Set<MaterialState> disabled = <MaterialState>{ MaterialState.disabled };
const Set<MaterialState> hovered = <MaterialState>{ MaterialState.hovered };
const Set<MaterialState> focused = <MaterialState>{ MaterialState.focused };
const Set<MaterialState> pressed = <MaterialState>{ MaterialState.pressed };
void checkButton(WidgetTester tester) {
final Material material = tester.widget<Material>(findMaterial);
final InkWell inkWell = tester.widget<InkWell>(findInkWell);
expect(material.textStyle!.color, foregroundColor);
expect(material.textStyle!.fontSize, 12);
expect(material.color, backgroundColor);
expect(material.shadowColor, shadowColor);
expect(material.elevation, elevation);
expect(MaterialStateProperty.resolveAs<MouseCursor>(inkWell.mouseCursor!, enabled), enabledMouseCursor);
expect(MaterialStateProperty.resolveAs<MouseCursor>(inkWell.mouseCursor!, disabled), disabledMouseCursor);
expect(inkWell.overlayColor!.resolve(hovered), foregroundColor.withOpacity(0.08));
expect(inkWell.overlayColor!.resolve(focused), foregroundColor.withOpacity(0.1));
expect(inkWell.overlayColor!.resolve(pressed), foregroundColor.withOpacity(0.1));
expect(inkWell.enableFeedback, enableFeedback);
expect(material.borderRadius, null);
expect(material.shape, shape);
expect(material.animationDuration, animationDuration);
expect(tester.getSize(find.byType(FilledButton)), const Size(200, 200));
final Align align = tester.firstWidget<Align>(find.ancestor(of: find.text('button'), matching: find.byType(Align)));
expect(align.alignment, alignment);
}
testWidgets('Button style overrides defaults', (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(buttonStyle: style));
await tester.pumpAndSettle(); // allow the animations to finish
checkButton(tester);
});
testWidgets('Button theme style overrides defaults', (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(themeStyle: style));
await tester.pumpAndSettle();
checkButton(tester);
});
testWidgets('Overall Theme button theme style overrides defaults', (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(overallStyle: style));
await tester.pumpAndSettle();
checkButton(tester);
});
// Same as the previous tests with empty ButtonStyle's instead of null.
testWidgets('Button style overrides defaults, empty theme and overall styles', (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(buttonStyle: style, themeStyle: const ButtonStyle(), overallStyle: const ButtonStyle()));
await tester.pumpAndSettle(); // allow the animations to finish
checkButton(tester);
});
testWidgets('Button theme style overrides defaults, empty button and overall styles', (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(buttonStyle: const ButtonStyle(), themeStyle: style, overallStyle: const ButtonStyle()));
await tester.pumpAndSettle(); // allow the animations to finish
checkButton(tester);
});
testWidgets('Overall Theme button theme style overrides defaults, null theme and empty overall style', (WidgetTester tester) async {
await tester.pumpWidget(buildFrame(buttonStyle: const ButtonStyle(), overallStyle: style));
await tester.pumpAndSettle(); // allow the animations to finish
checkButton(tester);
});
});
testWidgets('Theme shadowColor', (WidgetTester tester) async {
const ColorScheme colorScheme = ColorScheme.light();
const Color shadowColor = Color(0xff000001);
const Color overriddenColor = Color(0xff000002);
Widget buildFrame({ Color? overallShadowColor, Color? themeShadowColor, Color? shadowColor }) {
return MaterialApp(
theme: ThemeData.from(colorScheme: colorScheme).copyWith(
shadowColor: overallShadowColor,
),
home: Scaffold(
body: Center(
child: FilledButtonTheme(
data: FilledButtonThemeData(
style: FilledButton.styleFrom(
shadowColor: themeShadowColor,
),
),
child: Builder(
builder: (BuildContext context) {
return FilledButton(
style: FilledButton.styleFrom(
shadowColor: shadowColor,
),
onPressed: () { },
child: const Text('button'),
);
},
),
),
),
),
);
}
final Finder buttonMaterialFinder = find.descendant(
of: find.byType(FilledButton),
matching: find.byType(Material),
);
await tester.pumpWidget(buildFrame());
Material material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, Colors.black); //default
await tester.pumpWidget(buildFrame(themeShadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
await tester.pumpWidget(buildFrame(shadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
await tester.pumpWidget(buildFrame(overallShadowColor: overriddenColor, themeShadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
await tester.pumpWidget(buildFrame(themeShadowColor: overriddenColor, shadowColor: shadowColor));
await tester.pumpAndSettle(); // theme animation
material = tester.widget<Material>(buttonMaterialFinder);
expect(material.shadowColor, shadowColor);
});
}
| flutter/packages/flutter/test/material/filled_button_theme_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/filled_button_theme_test.dart",
"repo_id": "flutter",
"token_count": 3966
} | 691 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
class Page extends StatefulWidget {
const Page({
super.key,
required this.title,
required this.onDispose,
});
final String title;
final void Function()? onDispose;
@override
State<Page> createState() => _PageState();
}
class _PageState extends State<Page> {
@override
void dispose() {
widget.onDispose?.call();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Center(
child: FilledButton(
onPressed: () {},
child: Text(widget.title),
),
);
}
}
void main() {
// Regression test for https://github.com/flutter/flutter/issues/21506.
testWidgets('InkSplash receives textDirection', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Scaffold(
appBar: AppBar(title: const Text('Button Border Test')),
body: Center(
child: ElevatedButton(
child: const Text('Test'),
onPressed: () { },
),
),
),
));
await tester.tap(find.text('Test'));
// start ink animation which asserts for a textDirection.
await tester.pumpAndSettle(const Duration(milliseconds: 30));
expect(tester.takeException(), isNull);
});
testWidgets('Material2 - InkWell with NoSplash splashFactory paints nothing', (WidgetTester tester) async {
Widget buildFrame({ InteractiveInkFeatureFactory? splashFactory }) {
return MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Scaffold(
body: Center(
child: Material(
child: InkWell(
splashFactory: splashFactory,
onTap: () { },
child: const Text('test'),
),
),
),
),
);
}
// NoSplash.splashFactory, no splash circles drawn
await tester.pumpWidget(buildFrame(splashFactory: NoSplash.splashFactory));
{
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.text('test')));
final MaterialInkController material = Material.of(tester.element(find.text('test')));
await tester.pump(const Duration(milliseconds: 200));
expect(material, paintsExactlyCountTimes(#drawCircle, 0));
await gesture.up();
await tester.pumpAndSettle();
}
// Default splashFactory (from Theme.of().splashFactory), one splash circle drawn.
await tester.pumpWidget(buildFrame());
{
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.text('test')));
final MaterialInkController material = Material.of(tester.element(find.text('test')));
await tester.pump(const Duration(milliseconds: 200));
expect(material, paintsExactlyCountTimes(#drawCircle, 1));
await gesture.up();
await tester.pumpAndSettle();
}
});
testWidgets('Material3 - InkWell with NoSplash splashFactory paints nothing', (WidgetTester tester) async {
Widget buildFrame({ InteractiveInkFeatureFactory? splashFactory }) {
return MaterialApp(
home: Scaffold(
body: Center(
child: Material(
child: InkWell(
splashFactory: splashFactory,
onTap: () { },
child: const Text('test'),
),
),
),
),
);
}
// NoSplash.splashFactory, one rect is drawn for the highlight.
await tester.pumpWidget(buildFrame(splashFactory: NoSplash.splashFactory));
{
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.text('test')));
final MaterialInkController material = Material.of(tester.element(find.text('test')));
await tester.pump(const Duration(milliseconds: 200));
expect(material, paintsExactlyCountTimes(#drawRect, 1));
await gesture.up();
await tester.pumpAndSettle();
}
// Default splashFactory (from Theme.of().splashFactory), two rects are drawn for the splash and highlight.
await tester.pumpWidget(buildFrame());
{
final TestGesture gesture = await tester.startGesture(tester.getCenter(find.text('test')));
final MaterialInkController material = Material.of(tester.element(find.text('test')));
await tester.pump(const Duration(milliseconds: 200));
expect(material, paintsExactlyCountTimes(#drawRect, (kIsWeb && isCanvasKit ? 1 : 2)));
await gesture.up();
await tester.pumpAndSettle();
}
}, skip: kIsWeb && !isCanvasKit); // https://github.com/flutter/flutter/issues/99933
// Regression test for https://github.com/flutter/flutter/issues/136441.
testWidgets('PageView item can dispose when widget with NoSplash.splashFactory is tapped', (WidgetTester tester) async {
final PageController controller = PageController();
final List<int> disposedPageIndexes = <int>[];
await tester.pumpWidget(MaterialApp(
theme: ThemeData(splashFactory: NoSplash.splashFactory),
home: Scaffold(
body: PageView.builder(
controller: controller,
itemBuilder: (BuildContext context, int index) {
return Page(
title: 'Page $index',
onDispose: () {
disposedPageIndexes.add(index);
},
);
},
itemCount: 3,
),
),
));
controller.jumpToPage(1);
await tester.pumpAndSettle();
await tester.tap(find.text('Page 1'));
await tester.pumpAndSettle();
controller.jumpToPage(0);
await tester.pumpAndSettle();
expect(disposedPageIndexes, <int>[0, 1]);
controller.dispose();
});
}
| flutter/packages/flutter/test/material/ink_splash_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/ink_splash_test.dart",
"repo_id": "flutter",
"token_count": 2366
} | 692 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
// Pumps and ensures that the BottomSheet animates non-linearly.
Future<void> checkNonLinearAnimation(WidgetTester tester) async {
final Offset firstPosition = tester.getCenter(find.text('One'));
await tester.pump(const Duration(milliseconds: 30));
final Offset secondPosition = tester.getCenter(find.text('One'));
await tester.pump(const Duration(milliseconds: 30));
final Offset thirdPosition = tester.getCenter(find.text('One'));
final double dyDelta1 = secondPosition.dy - firstPosition.dy;
final double dyDelta2 = thirdPosition.dy - secondPosition.dy;
// If the animation were linear, these two values would be the same.
expect(dyDelta1, isNot(moreOrLessEquals(dyDelta2, epsilon: 0.1)));
}
testWidgets('Persistent draggableScrollableSheet localHistoryEntries test', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/110123
Widget buildFrame(Widget? bottomSheet) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(),
body: const Center(child: Text('body')),
bottomSheet: bottomSheet,
floatingActionButton: const FloatingActionButton(
onPressed: null,
child: Text('fab'),
),
),
);
}
final Widget draggableScrollableSheet = DraggableScrollableSheet(
expand: false,
snap: true,
initialChildSize: 0.3,
minChildSize: 0.3,
builder: (_, ScrollController controller) {
return ListView.builder(
itemExtent: 50.0,
itemCount: 50,
itemBuilder: (_, int index) => Text('Item $index'),
controller: controller,
);
},
);
await tester.pumpWidget(buildFrame(draggableScrollableSheet));
await tester.pumpAndSettle();
expect(find.byType(BackButton).hitTestable(), findsNothing);
await tester.drag(find.text('Item 2'), const Offset(0, -200.0));
await tester.pumpAndSettle();
// We've started to drag up, we should have a back button now for a11y
expect(find.byType(BackButton).hitTestable(), findsOneWidget);
await tester.fling(find.text('Item 2'), const Offset(0, 200.0), 2000.0);
await tester.pumpAndSettle();
// BackButton should be hidden
expect(find.byType(BackButton).hitTestable(), findsNothing);
// Show the back button again
await tester.drag(find.text('Item 2'), const Offset(0, -200.0));
await tester.pumpAndSettle();
expect(find.byType(BackButton).hitTestable(), findsOneWidget);
// Remove the draggableScrollableSheet should hide the back button
await tester.pumpWidget(buildFrame(null));
expect(find.byType(BackButton).hitTestable(), findsNothing);
});
// Regression test for https://github.com/flutter/flutter/issues/83668
testWidgets('Scaffold.bottomSheet update test', (WidgetTester tester) async {
Widget buildFrame(Widget? bottomSheet) {
return MaterialApp(
home: Scaffold(
body: const Placeholder(),
bottomSheet: bottomSheet,
),
);
}
await tester.pumpWidget(buildFrame(const Text('I love Flutter!')));
await tester.pumpWidget(buildFrame(null));
// The disappearing animation has not yet been completed.
await tester.pumpWidget(buildFrame(const Text('I love Flutter!')));
});
testWidgets('Verify that a BottomSheet can be rebuilt with ScaffoldFeatureController.setState()', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
int buildCount = 0;
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
final PersistentBottomSheetController bottomSheet = scaffoldKey.currentState!.showBottomSheet((_) {
return Builder(
builder: (BuildContext context) {
buildCount += 1;
return Container(height: 200.0);
},
);
});
await tester.pump();
expect(buildCount, equals(1));
bottomSheet.setState!(() { });
await tester.pump();
expect(buildCount, equals(2));
});
testWidgets('Verify that a persistent BottomSheet cannot be dismissed', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: const Center(child: Text('body')),
bottomSheet: DraggableScrollableSheet(
expand: false,
builder: (_, ScrollController controller) {
return ListView(
controller: controller,
shrinkWrap: true,
children: const <Widget>[
SizedBox(height: 100.0, child: Text('One')),
SizedBox(height: 100.0, child: Text('Two')),
SizedBox(height: 100.0, child: Text('Three')),
],
);
},
),
),
));
await tester.pumpAndSettle();
expect(find.text('Two'), findsOneWidget);
await tester.drag(find.text('Two'), const Offset(0.0, 400.0));
await tester.pumpAndSettle();
expect(find.text('Two'), findsOneWidget);
});
testWidgets('Verify that a scrollable BottomSheet can be dismissed', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
scaffoldKey.currentState!.showBottomSheet((BuildContext context) {
return ListView(
shrinkWrap: true,
primary: false,
children: const <Widget>[
SizedBox(height: 100.0, child: Text('One')),
SizedBox(height: 100.0, child: Text('Two')),
SizedBox(height: 100.0, child: Text('Three')),
],
);
});
await tester.pumpAndSettle();
expect(find.text('Two'), findsOneWidget);
await tester.drag(find.text('Two'), const Offset(0.0, 400.0));
await tester.pumpAndSettle();
expect(find.text('Two'), findsNothing);
});
testWidgets('Verify DraggableScrollableSheet.shouldCloseOnMinExtent == false prevents dismissal', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
scaffoldKey.currentState!.showBottomSheet((BuildContext context) {
return DraggableScrollableSheet(
expand: false,
shouldCloseOnMinExtent: false,
builder: (_, ScrollController controller) {
return ListView(
controller: controller,
shrinkWrap: true,
children: const <Widget>[
SizedBox(height: 100.0, child: Text('One')),
SizedBox(height: 100.0, child: Text('Two')),
SizedBox(height: 100.0, child: Text('Three')),
],
);
},
);
});
await tester.pumpAndSettle();
expect(find.text('Two'), findsOneWidget);
await tester.drag(find.text('Two'), const Offset(0.0, 400.0));
await tester.pumpAndSettle();
expect(find.text('Two'), findsOneWidget);
});
testWidgets('Verify that a BottomSheet animates non-linearly', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
scaffoldKey.currentState!.showBottomSheet((BuildContext context) {
return ListView(
shrinkWrap: true,
primary: false,
children: const <Widget>[
SizedBox(height: 100.0, child: Text('One')),
SizedBox(height: 100.0, child: Text('Two')),
SizedBox(height: 100.0, child: Text('Three')),
],
);
});
await tester.pump();
await checkNonLinearAnimation(tester);
await tester.pumpAndSettle();
expect(find.text('Two'), findsOneWidget);
await tester.drag(find.text('Two'), const Offset(0.0, 200.0));
await checkNonLinearAnimation(tester);
await tester.pumpAndSettle();
expect(find.text('Two'), findsNothing);
});
testWidgets('Verify that a scrollControlled BottomSheet can be dismissed', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
scaffoldKey.currentState!.showBottomSheet(
(BuildContext context) {
return DraggableScrollableSheet(
expand: false,
builder: (_, ScrollController controller) {
return ListView(
shrinkWrap: true,
controller: controller,
children: const <Widget>[
SizedBox(height: 100.0, child: Text('One')),
SizedBox(height: 100.0, child: Text('Two')),
SizedBox(height: 100.0, child: Text('Three')),
],
);
},
);
},
);
await tester.pumpAndSettle();
expect(find.text('Two'), findsOneWidget);
await tester.drag(find.text('Two'), const Offset(0.0, 400.0));
await tester.pumpAndSettle();
expect(find.text('Two'), findsNothing);
});
testWidgets('Verify that a persistent BottomSheet can fling up and hide the fab', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
appBar: AppBar(),
body: const Center(child: Text('body')),
bottomSheet: DraggableScrollableSheet(
expand: false,
builder: (_, ScrollController controller) {
return ListView.builder(
itemExtent: 50.0,
itemCount: 50,
itemBuilder: (_, int index) => Text('Item $index'),
controller: controller,
);
},
),
floatingActionButton: const FloatingActionButton(
onPressed: null,
child: Text('fab'),
),
),
),
);
await tester.pumpAndSettle();
expect(find.text('Item 2'), findsOneWidget);
expect(find.text('Item 22'), findsNothing);
expect(find.byType(FloatingActionButton), findsOneWidget);
expect(find.byType(FloatingActionButton).hitTestable(), findsOneWidget);
expect(find.byType(BackButton).hitTestable(), findsNothing);
await tester.drag(find.text('Item 2'), const Offset(0, -20.0));
await tester.pumpAndSettle();
expect(find.text('Item 2'), findsOneWidget);
expect(find.text('Item 22'), findsNothing);
expect(find.byType(FloatingActionButton), findsOneWidget);
expect(find.byType(FloatingActionButton).hitTestable(), findsOneWidget);
await tester.fling(find.text('Item 2'), const Offset(0.0, -600.0), 2000.0);
await tester.pumpAndSettle();
expect(find.text('Item 2'), findsNothing);
expect(find.text('Item 22'), findsOneWidget);
expect(find.byType(FloatingActionButton), findsOneWidget);
expect(find.byType(FloatingActionButton).hitTestable(), findsNothing);
});
testWidgets('Verify that a back button resets a persistent BottomSheet', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
appBar: AppBar(),
body: const Center(child: Text('body')),
bottomSheet: DraggableScrollableSheet(
expand: false,
builder: (_, ScrollController controller) {
return ListView.builder(
itemExtent: 50.0,
itemCount: 50,
itemBuilder: (_, int index) => Text('Item $index'),
controller: controller,
);
},
),
floatingActionButton: const FloatingActionButton(
onPressed: null,
child: Text('fab'),
),
),
),
);
await tester.pumpAndSettle();
expect(find.text('Item 2'), findsOneWidget);
expect(find.text('Item 22'), findsNothing);
expect(find.byType(BackButton).hitTestable(), findsNothing);
await tester.drag(find.text('Item 2'), const Offset(0, -20.0));
await tester.pumpAndSettle();
expect(find.text('Item 2'), findsOneWidget);
expect(find.text('Item 22'), findsNothing);
// We've started to drag up, we should have a back button now for a11y
expect(find.byType(BackButton).hitTestable(), findsOneWidget);
await tester.tap(find.byType(BackButton));
await tester.pumpAndSettle();
expect(find.byType(BackButton).hitTestable(), findsNothing);
expect(find.text('Item 2'), findsOneWidget);
expect(find.text('Item 22'), findsNothing);
await tester.fling(find.text('Item 2'), const Offset(0.0, -600.0), 2000.0);
await tester.pumpAndSettle();
expect(find.text('Item 2'), findsNothing);
expect(find.text('Item 22'), findsOneWidget);
expect(find.byType(BackButton).hitTestable(), findsOneWidget);
await tester.tap(find.byType(BackButton));
await tester.pumpAndSettle();
expect(find.byType(BackButton).hitTestable(), findsNothing);
expect(find.text('Item 2'), findsOneWidget);
expect(find.text('Item 22'), findsNothing);
});
testWidgets('Verify that a scrollable BottomSheet hides the fab when scrolled up', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
floatingActionButton: const FloatingActionButton(
onPressed: null,
child: Text('fab'),
),
),
));
scaffoldKey.currentState!.showBottomSheet(
(BuildContext context) {
return DraggableScrollableSheet(
expand: false,
builder: (_, ScrollController controller) {
return ListView(
controller: controller,
shrinkWrap: true,
children: const <Widget>[
SizedBox(height: 100.0, child: Text('One')),
SizedBox(height: 100.0, child: Text('Two')),
SizedBox(height: 100.0, child: Text('Three')),
SizedBox(height: 100.0, child: Text('Three')),
SizedBox(height: 100.0, child: Text('Three')),
SizedBox(height: 100.0, child: Text('Three')),
SizedBox(height: 100.0, child: Text('Three')),
SizedBox(height: 100.0, child: Text('Three')),
SizedBox(height: 100.0, child: Text('Three')),
SizedBox(height: 100.0, child: Text('Three')),
SizedBox(height: 100.0, child: Text('Three')),
],
);
},
);
},
);
await tester.pumpAndSettle();
expect(find.text('Two'), findsOneWidget);
expect(find.byType(FloatingActionButton).hitTestable(), findsOneWidget);
await tester.drag(find.text('Two'), const Offset(0.0, -600.0));
await tester.pumpAndSettle();
expect(find.text('Two'), findsOneWidget);
expect(find.byType(FloatingActionButton), findsOneWidget);
expect(find.byType(FloatingActionButton).hitTestable(), findsNothing);
});
testWidgets('showBottomSheet()', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
await tester.pumpWidget(MaterialApp(
home: Scaffold(
body: Placeholder(key: key),
),
));
int buildCount = 0;
showBottomSheet(
context: key.currentContext!,
builder: (BuildContext context) {
return Builder(
builder: (BuildContext context) {
buildCount += 1;
return Container(height: 200.0);
},
);
},
);
await tester.pump();
expect(buildCount, equals(1));
});
testWidgets('Scaffold removes top MediaQuery padding', (WidgetTester tester) async {
late BuildContext scaffoldContext;
late BuildContext bottomSheetContext;
await tester.pumpWidget(MaterialApp(
home: MediaQuery(
data: const MediaQueryData(
padding: EdgeInsets.all(50.0),
),
child: Scaffold(
resizeToAvoidBottomInset: false,
body: Builder(
builder: (BuildContext context) {
scaffoldContext = context;
return Container();
},
),
),
),
));
await tester.pump();
showBottomSheet(
context: scaffoldContext,
builder: (BuildContext context) {
bottomSheetContext = context;
return Container();
},
);
await tester.pump();
expect(
MediaQuery.of(bottomSheetContext).padding,
const EdgeInsets.only(
bottom: 50.0,
left: 50.0,
right: 50.0,
),
);
});
testWidgets('Scaffold.bottomSheet', (WidgetTester tester) async {
final Key bottomSheetKey = UniqueKey();
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: false),
home: Scaffold(
body: const Placeholder(),
bottomSheet: Container(
key: bottomSheetKey,
alignment: Alignment.center,
height: 200.0,
child: Builder(
builder: (BuildContext context) {
return ElevatedButton(
child: const Text('showModalBottomSheet'),
onPressed: () {
showModalBottomSheet<void>(
context: context,
builder: (BuildContext context) => const Text('modal bottom sheet'),
);
},
);
},
),
),
),
),
);
expect(find.text('showModalBottomSheet'), findsOneWidget);
expect(tester.getSize(find.byKey(bottomSheetKey)), const Size(800.0, 200.0));
expect(tester.getTopLeft(find.byKey(bottomSheetKey)), const Offset(0.0, 400.0));
// Show the modal bottomSheet
await tester.tap(find.text('showModalBottomSheet'));
await tester.pumpAndSettle();
expect(find.text('modal bottom sheet'), findsOneWidget);
// Dismiss the modal bottomSheet by tapping above the sheet
await tester.tapAt(const Offset(20.0, 20.0));
await tester.pumpAndSettle();
expect(find.text('modal bottom sheet'), findsNothing);
expect(find.text('showModalBottomSheet'), findsOneWidget);
// Remove the persistent bottomSheet
await tester.pumpWidget(
const MaterialApp(
home: Scaffold(
body: Placeholder(),
),
),
);
await tester.pumpAndSettle();
expect(find.text('showModalBottomSheet'), findsNothing);
expect(find.byKey(bottomSheetKey), findsNothing);
});
// Regression test for https://github.com/flutter/flutter/issues/71435
testWidgets(
'Scaffold.bottomSheet should be updated without creating a new RO'
' when the new widget has the same key and type.',
(WidgetTester tester) async {
Widget buildFrame(String text) {
return MaterialApp(
home: Scaffold(
body: const Placeholder(),
bottomSheet: Text(text),
),
);
}
await tester.pumpWidget(buildFrame('I love Flutter!'));
final RenderParagraph renderBeforeUpdate = tester.renderObject(find.text('I love Flutter!'));
await tester.pumpWidget(buildFrame('Flutter is the best!'));
await tester.pumpAndSettle();
final RenderParagraph renderAfterUpdate = tester.renderObject(find.text('Flutter is the best!'));
expect(renderBeforeUpdate, renderAfterUpdate);
},
);
testWidgets('Verify that visual properties are passed through', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey<ScaffoldState>();
const Color color = Colors.pink;
const double elevation = 9.0;
const ShapeBorder shape = BeveledRectangleBorder(borderRadius: BorderRadius.all(Radius.circular(12)));
const Clip clipBehavior = Clip.antiAlias;
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
scaffoldKey.currentState!.showBottomSheet((BuildContext context) {
return ListView(
shrinkWrap: true,
primary: false,
children: const <Widget>[
SizedBox(height: 100.0, child: Text('One')),
SizedBox(height: 100.0, child: Text('Two')),
SizedBox(height: 100.0, child: Text('Three')),
],
);
}, backgroundColor: color, elevation: elevation, shape: shape, clipBehavior: clipBehavior);
await tester.pumpAndSettle();
final BottomSheet bottomSheet = tester.widget(find.byType(BottomSheet));
expect(bottomSheet.backgroundColor, color);
expect(bottomSheet.elevation, elevation);
expect(bottomSheet.shape, shape);
expect(bottomSheet.clipBehavior, clipBehavior);
});
testWidgets('PersistentBottomSheetController.close dismisses the bottom sheet', (WidgetTester tester) async {
final GlobalKey<ScaffoldState> scaffoldKey = GlobalKey();
await tester.pumpWidget(MaterialApp(
home: Scaffold(
key: scaffoldKey,
body: const Center(child: Text('body')),
),
));
final PersistentBottomSheetController bottomSheet = scaffoldKey.currentState!.showBottomSheet((_) {
return Builder(
builder: (BuildContext context) {
return Container(height: 200.0);
},
);
});
await tester.pump();
expect(find.byType(BottomSheet), findsOneWidget);
bottomSheet.close();
await tester.pump();
expect(find.byType(BottomSheet), findsNothing);
});
}
| flutter/packages/flutter/test/material/persistent_bottom_sheet_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/persistent_bottom_sheet_test.dart",
"repo_id": "flutter",
"token_count": 9308
} | 693 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui';
import 'package:flutter/foundation.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/semantics_tester.dart';
void main() {
// Returns the RenderEditable at the given index, or the first if not given.
RenderEditable findRenderEditable(WidgetTester tester, {int index = 0}) {
final RenderObject root = tester.renderObject(find.byType(EditableText).at(index));
expect(root, isNotNull);
late RenderEditable renderEditable;
void recursiveFinder(RenderObject child) {
if (child is RenderEditable) {
renderEditable = child;
return;
}
child.visitChildren(recursiveFinder);
}
root.visitChildren(recursiveFinder);
expect(renderEditable, isNotNull);
return renderEditable;
}
List<TextSelectionPoint> globalize(Iterable<TextSelectionPoint> points, RenderBox box) {
return points.map<TextSelectionPoint>((TextSelectionPoint point) {
return TextSelectionPoint(
box.localToGlobal(point.point),
point.direction,
);
}).toList();
}
Offset textOffsetToPosition(WidgetTester tester, int offset, {int index = 0}) {
final RenderEditable renderEditable = findRenderEditable(tester, index: index);
final List<TextSelectionPoint> endpoints = globalize(
renderEditable.getEndpointsForSelection(
TextSelection.collapsed(offset: offset),
),
renderEditable,
);
expect(endpoints.length, 1);
return endpoints[0].point + const Offset(kIsWeb? 1.0 : 0.0, -2.0);
}
testWidgets('SearchBar defaults', (WidgetTester tester) async {
final ThemeData theme = ThemeData(useMaterial3: true);
final ColorScheme colorScheme = theme.colorScheme;
await tester.pumpWidget(
MaterialApp(
theme: theme,
home: const Material(
child: SearchBar(
hintText: 'hint text',
)
),
),
);
final Finder searchBarMaterial = find.descendant(
of: find.byType(SearchBar),
matching: find.byType(Material),
);
final Material material = tester.widget<Material>(searchBarMaterial);
checkSearchBarDefaults(tester, colorScheme, material);
});
testWidgets('SearchBar respects controller property', (WidgetTester tester) async {
const String defaultText = 'default text';
final TextEditingController controller = TextEditingController(text: defaultText);
addTearDown(controller.dispose);
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SearchBar(
controller: controller,
),
),
),
);
expect(controller.value.text, defaultText);
expect(find.text(defaultText), findsOneWidget);
const String updatedText = 'updated text';
await tester.enterText(find.byType(SearchBar), updatedText);
expect(controller.value.text, updatedText);
expect(find.text(defaultText), findsNothing);
expect(find.text(updatedText), findsOneWidget);
});
testWidgets('SearchBar respects focusNode property', (WidgetTester tester) async {
final FocusNode node = FocusNode();
addTearDown(node.dispose);
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SearchBar(
focusNode: node,
),
),
),
);
expect(node.hasFocus, false);
node.requestFocus();
await tester.pump();
expect(node.hasFocus, true);
node.unfocus();
await tester.pump();
expect(node.hasFocus, false);
});
testWidgets('SearchBar focusNode is hot swappable', (WidgetTester tester) async {
final FocusNode node1 = FocusNode();
addTearDown(node1.dispose);
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SearchBar(
focusNode: node1,
),
),
),
);
expect(node1.hasFocus, isFalse);
node1.requestFocus();
await tester.pump();
expect(node1.hasFocus, isTrue);
node1.unfocus();
await tester.pump();
expect(node1.hasFocus, isFalse);
final FocusNode node2 = FocusNode();
addTearDown(node2.dispose);
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SearchBar(
focusNode: node2,
),
),
),
);
expect(node1.hasFocus, isFalse);
expect(node2.hasFocus, isFalse);
node2.requestFocus();
await tester.pump();
expect(node1.hasFocus, isFalse);
expect(node2.hasFocus, isTrue);
node2.unfocus();
await tester.pump();
expect(node1.hasFocus, isFalse);
expect(node2.hasFocus, isFalse);
await tester.pumpWidget(
const MaterialApp(
home: Material(
child: SearchBar(),
),
),
);
expect(node1.hasFocus, isFalse);
expect(node2.hasFocus, isFalse);
await tester.tap(find.byType(SearchBar));
await tester.pump();
expect(node1.hasFocus, isFalse);
expect(node2.hasFocus, isFalse);
});
testWidgets('SearchBar has correct default layout and padding LTR', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Center(
child: SearchBar(
leading: IconButton(
icon: const Icon(Icons.search),
onPressed: () {},
),
trailing: <Widget>[
IconButton(
icon: const Icon(Icons.menu),
onPressed: () {},
)
],
),
),
),
);
final Rect barRect = tester.getRect(find.byType(SearchBar));
expect(barRect.size, const Size(800.0, 56.0));
expect(barRect, equals(const Rect.fromLTRB(0.0, 272.0, 800.0, 328.0)));
final Rect leadingIcon = tester.getRect(find.widgetWithIcon(IconButton, Icons.search));
// Default left padding is 8.0, and icon button has 8.0 padding, so in total the padding between
// the edge of the bar and the icon of the button is 16.0, which matches the spec.
expect(leadingIcon.left, equals(barRect.left + 8.0));
final Rect textField = tester.getRect(find.byType(TextField));
expect(textField.left, equals(leadingIcon.right + 8.0));
final Rect trailingIcon = tester.getRect(find.widgetWithIcon(IconButton, Icons.menu));
expect(trailingIcon.left, equals(textField.right + 8.0));
expect(trailingIcon.right, equals(barRect.right - 8.0));
});
testWidgets('SearchBar has correct default layout and padding - RTL', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.rtl,
child: Center(
child: SearchBar(
leading: IconButton(
icon: const Icon(Icons.search),
onPressed: () {},
),
trailing: <Widget>[
IconButton(
icon: const Icon(Icons.menu),
onPressed: () {},
)
],
),
),
),
),
);
final Rect barRect = tester.getRect(find.byType(SearchBar));
expect(barRect.size, const Size(800.0, 56.0));
expect(barRect, equals(const Rect.fromLTRB(0.0, 272.0, 800.0, 328.0)));
// The default padding is set to 8.0 so the distance between the icon of the button
// and the edge of the bar is 16.0, which matches the spec.
final Rect leadingIcon = tester.getRect(find.widgetWithIcon(IconButton, Icons.search));
expect(leadingIcon.right, equals(barRect.right - 8.0));
final Rect textField = tester.getRect(find.byType(TextField));
expect(textField.right, equals(leadingIcon.left - 8.0));
final Rect trailingIcon = tester.getRect(find.widgetWithIcon(IconButton, Icons.menu));
expect(trailingIcon.right, equals(textField.left - 8.0));
expect(trailingIcon.left, equals(barRect.left + 8.0));
});
testWidgets('SearchBar respects hintText property', (WidgetTester tester) async {
const String hintText = 'hint text';
await tester.pumpWidget(
const MaterialApp(
home: Material(
child: SearchBar(
hintText: hintText,
),
),
),
);
expect(find.text(hintText), findsOneWidget);
});
testWidgets('SearchBar respects leading property', (WidgetTester tester) async {
final ThemeData theme = ThemeData();
final ColorScheme colorScheme = theme.colorScheme;
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SearchBar(
leading: IconButton(
icon: const Icon(Icons.search),
onPressed: () {},
),
),
),
),
);
expect(find.widgetWithIcon(IconButton, Icons.search), findsOneWidget);
final Color? iconColor = _iconStyle(tester, Icons.search)?.color;
expect(iconColor, colorScheme.onSurface); // Default icon color.
});
testWidgets('SearchBar respects trailing property', (WidgetTester tester) async {
final ThemeData theme = ThemeData();
final ColorScheme colorScheme = theme.colorScheme;
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SearchBar(
trailing: <Widget>[
IconButton(
icon: const Icon(Icons.menu),
onPressed: () {},
),
],
),
),
),
);
expect(find.widgetWithIcon(IconButton, Icons.menu), findsOneWidget);
final Color? iconColor = _iconStyle(tester, Icons.menu)?.color;
expect(iconColor, colorScheme.onSurfaceVariant); // Default icon color.
});
testWidgets('SearchBar respects onTap property', (WidgetTester tester) async {
int tapCount = 0;
await tester.pumpWidget(
MaterialApp(
home: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Material(
child: SearchBar(
onTap: () {
setState(() {
tapCount++;
});
}
),
);
}
),
),
);
expect(tapCount, 0);
await tester.tap(find.byType(SearchBar));
expect(tapCount, 1);
await tester.tap(find.byType(SearchBar));
expect(tapCount, 2);
});
testWidgets('SearchBar respects onChanged property', (WidgetTester tester) async {
int changeCount = 0;
await tester.pumpWidget(
MaterialApp(
home: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Material(
child: SearchBar(
onChanged: (_) {
setState(() {
changeCount++;
});
}
),
);
}
),
),
);
expect(changeCount, 0);
await tester.enterText(find.byType(SearchBar), 'a');
expect(changeCount, 1);
await tester.enterText(find.byType(SearchBar), 'b');
expect(changeCount, 2);
});
testWidgets('SearchBar respects onSubmitted property', (WidgetTester tester) async {
String submittedQuery = '';
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SearchBar(
onSubmitted: (String text) {
submittedQuery = text;
},
),
),
),
);
await tester.enterText(find.byType(SearchBar), 'query');
await tester.testTextInput.receiveAction(TextInputAction.done);
expect(submittedQuery, equals('query'));
});
testWidgets('SearchBar respects constraints property', (WidgetTester tester) async {
const BoxConstraints constraints = BoxConstraints(maxWidth: 350.0, minHeight: 80);
await tester.pumpWidget(
const MaterialApp(
home: Center(
child: Material(
child: SearchBar(
constraints: constraints,
),
),
),
),
);
final Rect barRect = tester.getRect(find.byType(SearchBar));
expect(barRect.size, const Size(350.0, 80.0));
});
testWidgets('SearchBar respects elevation property', (WidgetTester tester) async {
const double pressedElevation = 0.0;
const double hoveredElevation = 1.0;
const double focusedElevation = 2.0;
const double defaultElevation = 3.0;
double getElevation(Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return pressedElevation;
}
if (states.contains(MaterialState.hovered)) {
return hoveredElevation;
}
if (states.contains(MaterialState.focused)) {
return focusedElevation;
}
return defaultElevation;
}
await tester.pumpWidget(
MaterialApp(
home: Center(
child: Material(
child: SearchBar(
elevation: MaterialStateProperty.resolveWith<double>(getElevation),
),
),
),
),
);
final Finder searchBarMaterial = find.descendant(
of: find.byType(SearchBar),
matching: find.byType(Material),
);
Material material = tester.widget<Material>(searchBarMaterial);
// On hovered.
final TestGesture gesture = await _pointGestureToSearchBar(tester);
await tester.pump();
material = tester.widget<Material>(searchBarMaterial);
expect(material.elevation, hoveredElevation);
// On pressed.
await gesture.down(tester.getCenter(find.byType(SearchBar)));
await tester.pumpAndSettle();
material = tester.widget<Material>(searchBarMaterial);
expect(material.elevation, pressedElevation);
// On focused.
await gesture.up();
await tester.pump();
// Remove the pointer so we are no longer hovering.
await gesture.removePointer();
await tester.pump();
material = tester.widget<Material>(searchBarMaterial);
expect(material.elevation, focusedElevation);
});
testWidgets('SearchBar respects backgroundColor property', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Center(
child: Material(
child: SearchBar(
backgroundColor: MaterialStateProperty.resolveWith<Color>(_getColor),
),
),
),
),
);
final Finder searchBarMaterial = find.descendant(
of: find.byType(SearchBar),
matching: find.byType(Material),
);
Material material = tester.widget<Material>(searchBarMaterial);
// On hovered.
final TestGesture gesture = await _pointGestureToSearchBar(tester);
await tester.pump();
material = tester.widget<Material>(searchBarMaterial);
expect(material.color, hoveredColor);
// On pressed.
await gesture.down(tester.getCenter(find.byType(SearchBar)));
await tester.pumpAndSettle();
material = tester.widget<Material>(searchBarMaterial);
expect(material.color, pressedColor);
// On focused.
await gesture.up();
await tester.pump();
// Remove the pointer so we are no longer hovering.
await gesture.removePointer();
await tester.pump();
material = tester.widget<Material>(searchBarMaterial);
expect(material.color, focusedColor);
});
testWidgets('SearchBar respects shadowColor property', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Center(
child: Material(
child: SearchBar(
shadowColor: MaterialStateProperty.resolveWith<Color>(_getColor),
),
),
),
),
);
final Finder searchBarMaterial = find.descendant(
of: find.byType(SearchBar),
matching: find.byType(Material),
);
Material material = tester.widget<Material>(searchBarMaterial);
// On hovered.
final TestGesture gesture = await _pointGestureToSearchBar(tester);
await tester.pump();
material = tester.widget<Material>(searchBarMaterial);
expect(material.shadowColor, hoveredColor);
// On pressed.
await gesture.down(tester.getCenter(find.byType(SearchBar)));
await tester.pumpAndSettle();
material = tester.widget<Material>(searchBarMaterial);
expect(material.shadowColor, pressedColor);
// On focused.
await gesture.up();
await tester.pump();
// Remove the pointer so we are no longer hovering.
await gesture.removePointer();
await tester.pump();
material = tester.widget<Material>(searchBarMaterial);
expect(material.shadowColor, focusedColor);
});
testWidgets('SearchBar respects surfaceTintColor property', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Center(
child: Material(
child: SearchBar(
surfaceTintColor: MaterialStateProperty.resolveWith<Color>(_getColor),
),
),
),
),
);
final Finder searchBarMaterial = find.descendant(
of: find.byType(SearchBar),
matching: find.byType(Material),
);
Material material = tester.widget<Material>(searchBarMaterial);
// On hovered.
final TestGesture gesture = await _pointGestureToSearchBar(tester);
await tester.pump();
material = tester.widget<Material>(searchBarMaterial);
expect(material.surfaceTintColor, hoveredColor);
// On pressed.
await gesture.down(tester.getCenter(find.byType(SearchBar)));
await tester.pumpAndSettle();
material = tester.widget<Material>(searchBarMaterial);
expect(material.surfaceTintColor, pressedColor);
// On focused.
await gesture.up();
await tester.pump();
// Remove the pointer so we are no longer hovering.
await gesture.removePointer();
await tester.pump();
material = tester.widget<Material>(searchBarMaterial);
expect(material.surfaceTintColor, focusedColor);
});
testWidgets('SearchBar respects overlayColor property', (WidgetTester tester) async {
final FocusNode focusNode = FocusNode();
addTearDown(focusNode.dispose);
await tester.pumpWidget(
MaterialApp(
home: Center(
child: Material(
child: SearchBar(
focusNode: focusNode,
overlayColor: MaterialStateProperty.resolveWith<Color>(_getColor),
),
),
),
),
);
RenderObject inkFeatures = tester.allRenderObjects.firstWhere((RenderObject object) => object.runtimeType.toString() == '_RenderInkFeatures');
// On hovered.
final TestGesture gesture = await _pointGestureToSearchBar(tester);
await tester.pumpAndSettle();
expect(inkFeatures, paints..rect(color: hoveredColor.withOpacity(1.0)));
// On pressed.
await tester.pumpAndSettle();
await gesture.down(tester.getCenter(find.byType(SearchBar)));
await tester.pumpAndSettle();
inkFeatures = tester.allRenderObjects.firstWhere((RenderObject object) => object.runtimeType.toString() == '_RenderInkFeatures');
expect(inkFeatures, paints..rect()..rect(color: pressedColor.withOpacity(1.0)));
// On focused.
await tester.pumpAndSettle();
await gesture.up();
await tester.pumpAndSettle();
// Remove the pointer so we are no longer hovering.
await gesture.removePointer();
await tester.pump();
inkFeatures = tester.allRenderObjects.firstWhere((RenderObject object) => object.runtimeType.toString() == '_RenderInkFeatures');
expect(inkFeatures, paints..rect()..rect(color: focusedColor.withOpacity(1.0)));
});
testWidgets('SearchBar respects side and shape properties', (WidgetTester tester) async {
const BorderSide pressedSide = BorderSide(width: 2.0);
const BorderSide hoveredSide = BorderSide(width: 3.0);
const BorderSide focusedSide = BorderSide(width: 4.0);
const BorderSide defaultSide = BorderSide(width: 5.0);
const OutlinedBorder pressedShape = RoundedRectangleBorder();
const OutlinedBorder hoveredShape = ContinuousRectangleBorder();
const OutlinedBorder focusedShape = CircleBorder();
const OutlinedBorder defaultShape = StadiumBorder();
BorderSide getSide(Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return pressedSide;
}
if (states.contains(MaterialState.hovered)) {
return hoveredSide;
}
if (states.contains(MaterialState.focused)) {
return focusedSide;
}
return defaultSide;
}
OutlinedBorder getShape(Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return pressedShape;
}
if (states.contains(MaterialState.hovered)) {
return hoveredShape;
}
if (states.contains(MaterialState.focused)) {
return focusedShape;
}
return defaultShape;
}
await tester.pumpWidget(
MaterialApp(
home: Center(
child: Material(
child: SearchBar(
side: MaterialStateProperty.resolveWith<BorderSide>(getSide),
shape: MaterialStateProperty.resolveWith<OutlinedBorder>(getShape),
),
),
),
),
);
final Finder searchBarMaterial = find.descendant(
of: find.byType(SearchBar),
matching: find.byType(Material),
);
Material material = tester.widget<Material>(searchBarMaterial);
// On hovered.
final TestGesture gesture = await _pointGestureToSearchBar(tester);
await tester.pump();
material = tester.widget<Material>(searchBarMaterial);
expect(material.shape, hoveredShape.copyWith(side: hoveredSide));
// On pressed.
await gesture.down(tester.getCenter(find.byType(SearchBar)));
await tester.pumpAndSettle();
material = tester.widget<Material>(searchBarMaterial);
expect(material.shape, pressedShape.copyWith(side: pressedSide));
// On focused.
await gesture.up();
await tester.pump();
// Remove the pointer so we are no longer hovering.
await gesture.removePointer();
await tester.pump();
material = tester.widget<Material>(searchBarMaterial);
expect(material.shape, focusedShape.copyWith(side: focusedSide));
});
testWidgets('SearchBar respects padding property', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Center(
child: Material(
child: SearchBar(
leading: Icon(Icons.search),
padding: MaterialStatePropertyAll<EdgeInsets>(EdgeInsets.all(16.0)),
trailing: <Widget>[
Icon(Icons.menu),
]
),
),
),
),
);
final Rect barRect = tester.getRect(find.byType(SearchBar));
final Rect leadingRect = tester.getRect(find.byIcon(Icons.search));
final Rect textFieldRect = tester.getRect(find.byType(TextField));
final Rect trailingRect = tester.getRect(find.byIcon(Icons.menu));
expect(barRect.left, leadingRect.left - 16.0);
expect(leadingRect.right, textFieldRect.left - 16.0);
expect(textFieldRect.right, trailingRect.left - 16.0);
expect(trailingRect.right, barRect.right - 16.0);
});
testWidgets('SearchBar respects hintStyle property', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Center(
child: Material(
child: SearchBar(
hintText: 'hint text',
hintStyle: MaterialStateProperty.resolveWith<TextStyle?>(_getTextStyle),
),
),
),
),
);
// On hovered.
final TestGesture gesture = await _pointGestureToSearchBar(tester);
await tester.pump();
Text helperText = tester.widget(find.text('hint text'));
expect(helperText.style?.color, hoveredColor);
// On pressed.
await gesture.down(tester.getCenter(find.byType(SearchBar)));
await tester.pumpAndSettle();
helperText = tester.widget(find.text('hint text'));
expect(helperText.style?.color, pressedColor);
// On focused.
await gesture.up();
await tester.pump();
// Remove the pointer so we are no longer hovering.
await gesture.removePointer();
await tester.pump();
helperText = tester.widget(find.text('hint text'));
expect(helperText.style?.color, focusedColor);
});
testWidgets('SearchBar respects textStyle property', (WidgetTester tester) async {
final TextEditingController controller = TextEditingController(text: 'input text');
addTearDown(controller.dispose);
await tester.pumpWidget(
MaterialApp(
home: Center(
child: Material(
child: SearchBar(
controller: controller,
textStyle: MaterialStateProperty.resolveWith<TextStyle?>(_getTextStyle),
),
),
),
),
);
// On hovered.
final TestGesture gesture = await _pointGestureToSearchBar(tester);
await tester.pump();
EditableText inputText = tester.widget(find.text('input text'));
expect(inputText.style.color, hoveredColor);
// On pressed.
await gesture.down(tester.getCenter(find.byType(SearchBar)));
await tester.pumpAndSettle();
inputText = tester.widget(find.text('input text'));
expect(inputText.style.color, pressedColor);
// On focused.
await gesture.up();
await tester.pump();
// Remove the pointer so we are no longer hovering.
await gesture.removePointer();
await tester.pump();
inputText = tester.widget(find.text('input text'));
expect(inputText.style.color, focusedColor);
});
testWidgets('SearchBar respects textCapitalization property', (WidgetTester tester) async {
Widget buildSearchBar(TextCapitalization textCapitalization) {
return MaterialApp(
home: Center(
child: Material(
child: SearchBar(
textCapitalization: textCapitalization,
),
),
),
);
}
await tester.pumpWidget(buildSearchBar(TextCapitalization.characters));
await tester.pump();
TextField textField = tester.widget(find.byType(TextField));
expect(textField.textCapitalization, TextCapitalization.characters);
await tester.pumpWidget(buildSearchBar(TextCapitalization.sentences));
await tester.pump();
textField = tester.widget(find.byType(TextField));
expect(textField.textCapitalization, TextCapitalization.sentences);
await tester.pumpWidget(buildSearchBar(TextCapitalization.words));
await tester.pump();
textField = tester.widget(find.byType(TextField));
expect(textField.textCapitalization, TextCapitalization.words);
await tester.pumpWidget(buildSearchBar(TextCapitalization.none));
await tester.pump();
textField = tester.widget(find.byType(TextField));
expect(textField.textCapitalization, TextCapitalization.none);
});
testWidgets('SearchAnchor respects textCapitalization property', (WidgetTester tester) async {
Widget buildSearchAnchor(TextCapitalization textCapitalization) {
return MaterialApp(
home: Center(
child: Material(
child: SearchAnchor(
textCapitalization: textCapitalization,
builder: (BuildContext context, SearchController controller) {
return IconButton(
icon: const Icon(Icons.ac_unit),
onPressed: () {
controller.openView();
},
);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
);
}
await tester.pumpWidget(buildSearchAnchor(TextCapitalization.characters));
await tester.pump();
await tester.tap(find.widgetWithIcon(IconButton, Icons.ac_unit));
await tester.pumpAndSettle();
TextField textField = tester.widget(find.byType(TextField));
expect(textField.textCapitalization, TextCapitalization.characters);
await tester.tap(find.widgetWithIcon(IconButton, Icons.arrow_back));
await tester.pump();
await tester.pumpWidget(buildSearchAnchor(TextCapitalization.none));
await tester.pump();
await tester.tap(find.widgetWithIcon(IconButton, Icons.ac_unit));
await tester.pumpAndSettle();
textField = tester.widget(find.byType(TextField));
expect(textField.textCapitalization, TextCapitalization.none);
});
testWidgets('SearchAnchor respects viewOnChanged and viewOnSubmitted properties', (WidgetTester tester) async {
final SearchController controller = SearchController();
addTearDown(controller.dispose);
int onChangedCalled = 0;
int onSubmittedCalled = 0;
await tester.pumpWidget(MaterialApp(
home: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Center(
child: Material(
child: SearchAnchor(
searchController: controller,
viewOnChanged: (String value) {
setState(() {
onChangedCalled = onChangedCalled + 1;
});
},
viewOnSubmitted: (String value) {
setState(() {
onSubmittedCalled = onSubmittedCalled + 1;
});
controller.closeView(value);
},
builder: (BuildContext context, SearchController controller) {
return SearchBar(
onTap: () {
if (!controller.isOpen) {
controller.openView();
}
},
);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
);
}
),
));
await tester.tap(find.byType(SearchBar)); // Open search view.
await tester.pumpAndSettle();
expect(controller.isOpen, true);
final Finder barOnView = find.descendant(
of: findViewContent(),
matching: find.byType(TextField)
);
await tester.enterText(barOnView, 'a');
expect(onChangedCalled, 1);
await tester.enterText(barOnView, 'abc');
expect(onChangedCalled, 2);
await tester.testTextInput.receiveAction(TextInputAction.done);
expect(onSubmittedCalled, 1);
expect(controller.isOpen, false);
});
testWidgets('SearchAnchor.bar respects textCapitalization property', (WidgetTester tester) async {
Widget buildSearchAnchor(TextCapitalization textCapitalization) {
return MaterialApp(
home: Center(
child: Material(
child: SearchAnchor.bar(
textCapitalization: textCapitalization,
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
);
}
await tester.pumpWidget(buildSearchAnchor(TextCapitalization.characters));
await tester.pump();
await tester.tap(find.byType(SearchBar)); // Open search view.
await tester.pumpAndSettle();
final Finder textFieldFinder = find.descendant(of: findViewContent(), matching: find.byType(TextField));
final TextField textFieldInView = tester.widget<TextField>(textFieldFinder);
expect(textFieldInView.textCapitalization, TextCapitalization.characters);
// Close search view.
await tester.tap(find.widgetWithIcon(IconButton, Icons.arrow_back));
await tester.pumpAndSettle();
final TextField textField = tester.widget(find.byType(TextField));
expect(textField.textCapitalization, TextCapitalization.characters);
});
testWidgets('SearchAnchor.bar respects onChanged and onSubmitted properties', (WidgetTester tester) async {
final SearchController controller = SearchController();
addTearDown(controller.dispose);
int onChangedCalled = 0;
int onSubmittedCalled = 0;
await tester.pumpWidget(MaterialApp(
home: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Center(
child: Material(
child: SearchAnchor.bar(
searchController: controller,
onSubmitted: (String value) {
setState(() {
onSubmittedCalled = onSubmittedCalled + 1;
});
controller.closeView(value);
},
onChanged: (String value) {
setState(() {
onChangedCalled = onChangedCalled + 1;
});
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
);
}
),
));
await tester.tap(find.byType(SearchBar)); // Open search view.
await tester.pumpAndSettle();
expect(controller.isOpen, true);
final Finder barOnView = find.descendant(
of: findViewContent(),
matching: find.byType(TextField)
);
await tester.enterText(barOnView, 'a');
expect(onChangedCalled, 1);
await tester.enterText(barOnView, 'abc');
expect(onChangedCalled, 2);
await tester.testTextInput.receiveAction(TextInputAction.done);
expect(onSubmittedCalled, 1);
expect(controller.isOpen, false);
await tester.testTextInput.receiveAction(TextInputAction.done);
expect(onSubmittedCalled, 2);
});
testWidgets('hintStyle can override textStyle for hintText', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Center(
child: Material(
child: SearchBar(
hintText: 'hint text',
hintStyle: MaterialStateProperty.resolveWith<TextStyle?>(_getTextStyle),
textStyle: const MaterialStatePropertyAll<TextStyle>(TextStyle(color: Colors.pink)),
),
),
),
),
);
// On hovered.
final TestGesture gesture = await _pointGestureToSearchBar(tester);
await tester.pump();
Text helperText = tester.widget(find.text('hint text'));
expect(helperText.style?.color, hoveredColor);
// On pressed.
await gesture.down(tester.getCenter(find.byType(SearchBar)));
await tester.pumpAndSettle();
helperText = tester.widget(find.text('hint text'));
expect(helperText.style?.color, pressedColor);
// On focused.
await gesture.up();
await tester.pump();
// Remove the pointer so we are no longer hovering.
await gesture.removePointer();
await tester.pump();
helperText = tester.widget(find.text('hint text'));
expect(helperText.style?.color, focusedColor);
});
// Regression test for https://github.com/flutter/flutter/issues/127092.
testWidgets('The text is still centered when SearchBar text field is smaller than 48', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(useMaterial3: true),
home: const Center(
child: Material(
child: SearchBar(
constraints: BoxConstraints.tightFor(height: 35.0),
),
),
),
),
);
await tester.enterText(find.byType(TextField), 'input text');
final Finder textContent = find.text('input text');
final double textCenterY = tester.getCenter(textContent).dy;
final Finder searchBar = find.byType(SearchBar);
final double searchBarCenterY = tester.getCenter(searchBar).dy;
expect(textCenterY, searchBarCenterY);
});
testWidgets('The search view defaults', (WidgetTester tester) async {
final ThemeData theme = ThemeData(useMaterial3: true);
final ColorScheme colorScheme = theme.colorScheme;
await tester.pumpWidget(
MaterialApp(
theme: theme,
home: Scaffold(
body: Material(
child: Align(
alignment: Alignment.topLeft,
child: SearchAnchor(
viewHintText: 'hint text',
builder: (BuildContext context, SearchController controller) {
return const Icon(Icons.search);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
),
),
);
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
final Material material = getSearchViewMaterial(tester);
expect(material.elevation, 6.0);
expect(material.color, colorScheme.surfaceContainerHigh);
expect(material.surfaceTintColor, Colors.transparent);
expect(material.clipBehavior, Clip.antiAlias);
final Finder findDivider = find.byType(Divider);
final Container dividerContainer = tester.widget<Container>(find.descendant(of: findDivider, matching: find.byType(Container)).first);
final BoxDecoration decoration = dividerContainer.decoration! as BoxDecoration;
expect(decoration.border!.bottom.color, colorScheme.outline);
// Default search view has a leading back button on the start of the header.
expect(find.widgetWithIcon(IconButton, Icons.arrow_back), findsOneWidget);
final Text helperText = tester.widget(find.text('hint text'));
expect(helperText.style?.color, colorScheme.onSurfaceVariant);
expect(helperText.style?.fontSize, 16.0);
expect(helperText.style?.fontFamily, 'Roboto');
expect(helperText.style?.fontWeight, FontWeight.w400);
const String input = 'entered text';
await tester.enterText(find.byType(SearchBar), input);
final EditableText inputText = tester.widget(find.text(input));
expect(inputText.style.color, colorScheme.onSurface);
expect(inputText.style.fontSize, 16.0);
expect(inputText.style.fontFamily, 'Roboto');
expect(inputText.style.fontWeight, FontWeight.w400);
});
testWidgets('The search view default size on different platforms', (WidgetTester tester) async {
// The search view should be is full-screen on mobile platforms,
// and have a size of (360, 2/3 screen height) on other platforms
Widget buildSearchAnchor(TargetPlatform platform) {
return MaterialApp(
theme: ThemeData(platform: platform),
home: Scaffold(
body: SafeArea(
child: Material(
child: Align(
alignment: Alignment.topLeft,
child: SearchAnchor(
builder: (BuildContext context, SearchController controller) {
return const Icon(Icons.search);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
),
),
);
}
for (final TargetPlatform platform in <TargetPlatform>[ TargetPlatform.iOS, TargetPlatform.android, TargetPlatform.fuchsia ]) {
await tester.pumpWidget(Container());
await tester.pumpWidget(buildSearchAnchor(platform));
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
final SizedBox sizedBox = tester.widget<SizedBox>(find.descendant(of: findViewContent(), matching: find.byType(SizedBox)).first);
expect(sizedBox.width, 800.0);
expect(sizedBox.height, 600.0);
}
for (final TargetPlatform platform in <TargetPlatform>[ TargetPlatform.linux, TargetPlatform.windows ]) {
await tester.pumpWidget(Container());
await tester.pumpWidget(buildSearchAnchor(platform));
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
final SizedBox sizedBox = tester.widget<SizedBox>(find.descendant(of: findViewContent(), matching: find.byType(SizedBox)).first);
expect(sizedBox.width, 360.0);
expect(sizedBox.height, 400.0);
}
});
testWidgets('SearchAnchor respects isFullScreen property', (WidgetTester tester) async {
Widget buildSearchAnchor(TargetPlatform platform) {
return MaterialApp(
theme: ThemeData(platform: platform),
home: Scaffold(
body: SafeArea(
child: Material(
child: Align(
alignment: Alignment.topLeft,
child: SearchAnchor(
isFullScreen: true,
builder: (BuildContext context, SearchController controller) {
return const Icon(Icons.search);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
),
),
);
}
for (final TargetPlatform platform in <TargetPlatform>[ TargetPlatform.linux, TargetPlatform.windows ]) {
await tester.pumpWidget(Container());
await tester.pumpWidget(buildSearchAnchor(platform));
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
final SizedBox sizedBox = tester.widget<SizedBox>(find.descendant(of: findViewContent(), matching: find.byType(SizedBox)).first);
expect(sizedBox.width, 800.0);
expect(sizedBox.height, 600.0);
}
});
testWidgets('SearchAnchor respects controller property', (WidgetTester tester) async {
const String defaultText = 'initial text';
final SearchController controller = SearchController();
addTearDown(controller.dispose);
controller.text = defaultText;
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SearchAnchor(
searchController: controller,
builder: (BuildContext context, SearchController controller) {
return IconButton(icon: const Icon(Icons.search), onPressed: () {
controller.openView();
},);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
);
await tester.tap(find.widgetWithIcon(IconButton, Icons.search));
await tester.pumpAndSettle();
expect(controller.value.text, defaultText);
expect(find.text(defaultText), findsOneWidget);
const String updatedText = 'updated text';
await tester.enterText(find.byType(SearchBar), updatedText);
expect(controller.value.text, updatedText);
expect(find.text(defaultText), findsNothing);
expect(find.text(updatedText), findsOneWidget);
});
testWidgets('SearchAnchor attaches and detaches controllers property', (WidgetTester tester) async {
Widget builder(BuildContext context, SearchController controller) {
return const Icon(Icons.search);
}
List<Widget> suggestionsBuilder(BuildContext context, SearchController controller) {
return const <Widget>[];
}
final SearchController controller1 = SearchController();
addTearDown(controller1.dispose);
expect(controller1.isAttached, isFalse);
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SearchAnchor(
searchController: controller1,
builder: builder,
suggestionsBuilder: suggestionsBuilder,
),
),
),
);
expect(controller1.isAttached, isTrue);
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SearchAnchor(
builder: builder,
suggestionsBuilder: suggestionsBuilder,
),
),
),
);
expect(controller1.isAttached, isFalse);
final SearchController controller2 = SearchController();
addTearDown(controller2.dispose);
expect(controller2.isAttached, isFalse);
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SearchAnchor(
searchController: controller2,
builder: builder,
suggestionsBuilder: suggestionsBuilder,
),
),
),
);
expect(controller1.isAttached, isFalse);
expect(controller2.isAttached, isTrue);
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SearchAnchor(
builder: builder,
suggestionsBuilder: suggestionsBuilder,
),
),
),
);
expect(controller1.isAttached, isFalse);
expect(controller2.isAttached, isFalse);
});
testWidgets('SearchAnchor respects viewBuilder property', (WidgetTester tester) async {
Widget buildAnchor({ViewBuilder? viewBuilder}) {
return MaterialApp(
home: Material(
child: SearchAnchor(
viewBuilder: viewBuilder,
builder: (BuildContext context, SearchController controller) {
return IconButton(icon: const Icon(Icons.search), onPressed: () {
controller.openView();
},);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
);
}
await tester.pumpWidget(buildAnchor());
await tester.tap(find.widgetWithIcon(IconButton, Icons.search));
await tester.pumpAndSettle();
// Default is a ListView.
expect(find.byType(ListView), findsOneWidget);
await tester.pumpWidget(Container());
await tester.pumpWidget(buildAnchor(viewBuilder: (Iterable<Widget> suggestions)
=> GridView.count(crossAxisCount: 5, children: suggestions.toList(),)
));
await tester.tap(find.widgetWithIcon(IconButton, Icons.search));
await tester.pumpAndSettle();
expect(find.byType(ListView), findsNothing);
expect(find.byType(GridView), findsOneWidget);
});
testWidgets('SearchAnchor respects viewLeading property', (WidgetTester tester) async {
Widget buildAnchor({Widget? viewLeading}) {
return MaterialApp(
home: Material(
child: SearchAnchor(
viewLeading: viewLeading,
builder: (BuildContext context, SearchController controller) {
return IconButton(icon: const Icon(Icons.search), onPressed: () {
controller.openView();
},);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
);
}
await tester.pumpWidget(buildAnchor());
await tester.tap(find.widgetWithIcon(IconButton, Icons.search));
await tester.pumpAndSettle();
// Default is a icon button with arrow_back.
expect(find.widgetWithIcon(IconButton, Icons.arrow_back), findsOneWidget);
await tester.pumpWidget(Container());
await tester.pumpWidget(buildAnchor(viewLeading: const Icon(Icons.history)));
await tester.tap(find.widgetWithIcon(IconButton, Icons.search));
await tester.pumpAndSettle();
expect(find.byIcon(Icons.arrow_back), findsNothing);
expect(find.byIcon(Icons.history), findsOneWidget);
});
testWidgets('SearchAnchor respects viewTrailing property', (WidgetTester tester) async {
Widget buildAnchor({Iterable<Widget>? viewTrailing}) {
return MaterialApp(
home: Material(
child: SearchAnchor(
viewTrailing: viewTrailing,
builder: (BuildContext context, SearchController controller) {
return IconButton(icon: const Icon(Icons.search), onPressed: () {
controller.openView();
},);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
);
}
await tester.pumpWidget(buildAnchor());
await tester.tap(find.widgetWithIcon(IconButton, Icons.search));
await tester.pumpAndSettle();
// Default is a icon button with close icon when input is not empty.
await tester.enterText(findTextField(), 'a');
await tester.pump();
expect(find.widgetWithIcon(IconButton, Icons.close), findsOneWidget);
await tester.pumpWidget(Container());
await tester.pumpWidget(buildAnchor(viewTrailing: <Widget>[const Icon(Icons.history)]));
await tester.tap(find.widgetWithIcon(IconButton, Icons.search));
await tester.pumpAndSettle();
expect(find.byIcon(Icons.close), findsNothing);
expect(find.byIcon(Icons.history), findsOneWidget);
});
testWidgets('SearchAnchor respects viewHintText property', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Material(
child: SearchAnchor(
viewHintText: 'hint text',
builder: (BuildContext context, SearchController controller) {
return IconButton(icon: const Icon(Icons.search), onPressed: () {
controller.openView();
},);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
));
await tester.tap(find.widgetWithIcon(IconButton, Icons.search));
await tester.pumpAndSettle();
expect(find.text('hint text'), findsOneWidget);
});
testWidgets('SearchAnchor respects viewBackgroundColor property', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Material(
child: SearchAnchor(
viewBackgroundColor: Colors.purple,
builder: (BuildContext context, SearchController controller) {
return IconButton(icon: const Icon(Icons.search), onPressed: () {
controller.openView();
},);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
));
await tester.tap(find.widgetWithIcon(IconButton, Icons.search));
await tester.pumpAndSettle();
expect(getSearchViewMaterial(tester).color, Colors.purple);
});
testWidgets('SearchAnchor respects viewElevation property', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Material(
child: SearchAnchor(
viewElevation: 3.0,
builder: (BuildContext context, SearchController controller) {
return IconButton(icon: const Icon(Icons.search), onPressed: () {
controller.openView();
},);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
));
await tester.tap(find.widgetWithIcon(IconButton, Icons.search));
await tester.pumpAndSettle();
expect(getSearchViewMaterial(tester).elevation, 3.0);
});
testWidgets('SearchAnchor respects viewSurfaceTint property', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Material(
child: SearchAnchor(
viewSurfaceTintColor: Colors.purple,
builder: (BuildContext context, SearchController controller) {
return IconButton(icon: const Icon(Icons.search), onPressed: () {
controller.openView();
},);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
));
await tester.tap(find.widgetWithIcon(IconButton, Icons.search));
await tester.pumpAndSettle();
expect(getSearchViewMaterial(tester).surfaceTintColor, Colors.purple);
});
testWidgets('SearchAnchor respects viewSide property', (WidgetTester tester) async {
const BorderSide side = BorderSide(color: Colors.purple, width: 5.0);
await tester.pumpWidget(MaterialApp(
home: Material(
child: SearchAnchor(
isFullScreen: false,
viewSide: side,
builder: (BuildContext context, SearchController controller) {
return IconButton(icon: const Icon(Icons.search), onPressed: () {
controller.openView();
},);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
));
await tester.tap(find.widgetWithIcon(IconButton, Icons.search));
await tester.pumpAndSettle();
expect(getSearchViewMaterial(tester).shape, RoundedRectangleBorder(side: side, borderRadius: BorderRadius.circular(28.0)));
});
testWidgets('SearchAnchor respects viewShape property', (WidgetTester tester) async {
const BorderSide side = BorderSide(color: Colors.purple, width: 5.0);
const OutlinedBorder shape = StadiumBorder(side: side);
await tester.pumpWidget(MaterialApp(
home: Material(
child: SearchAnchor(
isFullScreen: false,
viewShape: shape,
builder: (BuildContext context, SearchController controller) {
return IconButton(icon: const Icon(Icons.search), onPressed: () {
controller.openView();
},);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
));
await tester.tap(find.widgetWithIcon(IconButton, Icons.search));
await tester.pumpAndSettle();
expect(getSearchViewMaterial(tester).shape, shape);
});
testWidgets('SearchAnchor respects headerTextStyle property', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Material(
child: SearchAnchor(
headerTextStyle: theme.textTheme.bodyLarge?.copyWith(color: Colors.red),
builder: (BuildContext context, SearchController controller) {
return IconButton(icon: const Icon(Icons.search), onPressed: () {
controller.openView();
},);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
));
await tester.tap(find.widgetWithIcon(IconButton, Icons.search));
await tester.pumpAndSettle();
await tester.enterText(find.byType(SearchBar), 'input text');
await tester.pumpAndSettle();
final EditableText inputText = tester.widget(find.text('input text'));
expect(inputText.style.color, Colors.red);
});
testWidgets('SearchAnchor respects headerHintStyle property', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Material(
child: SearchAnchor(
viewHintText: 'hint text',
headerHintStyle: theme.textTheme.bodyLarge?.copyWith(color: Colors.orange),
builder: (BuildContext context, SearchController controller) {
return IconButton(icon: const Icon(Icons.search), onPressed: () {
controller.openView();
},);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
));
await tester.tap(find.widgetWithIcon(IconButton, Icons.search));
await tester.pumpAndSettle();
final Text inputText = tester.widget(find.text('hint text'));
expect(inputText.style?.color, Colors.orange);
});
testWidgets('SearchAnchor respects dividerColor property', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Material(
child: SearchAnchor(
dividerColor: Colors.red,
builder: (BuildContext context, SearchController controller) {
return IconButton(icon: const Icon(Icons.search), onPressed: () {
controller.openView();
},);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
));
await tester.tap(find.widgetWithIcon(IconButton, Icons.search));
await tester.pumpAndSettle();
final Finder findDivider = find.byType(Divider);
final Container dividerContainer = tester.widget<Container>(find.descendant(of: findDivider, matching: find.byType(Container)).first);
final BoxDecoration decoration = dividerContainer.decoration! as BoxDecoration;
expect(decoration.border!.bottom.color, Colors.red);
});
testWidgets('SearchAnchor respects viewConstraints property', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Material(
child: Center(
child: SearchAnchor(
isFullScreen: false,
viewConstraints: BoxConstraints.tight(const Size(280.0, 390.0)),
builder: (BuildContext context, SearchController controller) {
return IconButton(icon: const Icon(Icons.search), onPressed: () {
controller.openView();
},);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
));
await tester.tap(find.widgetWithIcon(IconButton, Icons.search));
await tester.pumpAndSettle();
final SizedBox sizedBox = tester.widget<SizedBox>(find.descendant(of: findViewContent(), matching: find.byType(SizedBox)).first);
expect(sizedBox.width, 280.0);
expect(sizedBox.height, 390.0);
});
testWidgets('SearchAnchor respects builder property - LTR', (WidgetTester tester) async {
Widget buildAnchor({required SearchAnchorChildBuilder builder}) {
return MaterialApp(
home: Material(
child: Align(
alignment: Alignment.topCenter,
child: SearchAnchor(
isFullScreen: false,
builder: builder,
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
);
}
await tester.pumpWidget(buildAnchor(
builder: (BuildContext context, SearchController controller)
=> const Icon(Icons.search)
));
final Rect anchorRect = tester.getRect(find.byIcon(Icons.search));
expect(anchorRect.size, const Size(24.0, 24.0));
expect(anchorRect, equals(const Rect.fromLTRB(388.0, 0.0, 412.0, 24.0)));
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
final Rect searchViewRect = tester.getRect(find.descendant(of: findViewContent(), matching: find.byType(SizedBox)).first);
expect(searchViewRect, equals(const Rect.fromLTRB(388.0, 0.0, 748.0, 400.0)));
// Search view top left should be the same as the anchor top left
expect(searchViewRect.topLeft, anchorRect.topLeft);
});
testWidgets('SearchAnchor respects builder property - RTL', (WidgetTester tester) async {
Widget buildAnchor({required SearchAnchorChildBuilder builder}) {
return MaterialApp(
home: Directionality(
textDirection: TextDirection.rtl,
child: Material(
child: Align(
alignment: Alignment.topCenter,
child: SearchAnchor(
isFullScreen: false,
builder: builder,
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
),
);
}
await tester.pumpWidget(buildAnchor(builder: (BuildContext context, SearchController controller)
=> const Icon(Icons.search)));
final Rect anchorRect = tester.getRect(find.byIcon(Icons.search));
expect(anchorRect.size, const Size(24.0, 24.0));
expect(anchorRect, equals(const Rect.fromLTRB(388.0, 0.0, 412.0, 24.0)));
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
final Rect searchViewRect = tester.getRect(find.descendant(of: findViewContent(), matching: find.byType(SizedBox)).first);
expect(searchViewRect, equals(const Rect.fromLTRB(52.0, 0.0, 412.0, 400.0)));
// Search view top right should be the same as the anchor top right
expect(searchViewRect.topRight, anchorRect.topRight);
});
testWidgets('SearchAnchor respects suggestionsBuilder property', (WidgetTester tester) async {
final SearchController controller = SearchController();
addTearDown(controller.dispose);
const String suggestion = 'suggestion text';
await tester.pumpWidget(MaterialApp(
home: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Material(
child: Align(
alignment: Alignment.topCenter,
child: SearchAnchor(
searchController: controller,
builder: (BuildContext context, SearchController controller) {
return const Icon(Icons.search);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[
ListTile(
title: const Text(suggestion),
onTap: () {
setState(() {
controller.closeView(suggestion);
});
}),
];
},
),
),
);
}
),
));
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
final Finder listTile = find.widgetWithText(ListTile, suggestion);
expect(listTile, findsOneWidget);
await tester.tap(listTile);
await tester.pumpAndSettle();
expect(controller.isOpen, false);
expect(controller.value.text, suggestion);
});
testWidgets('SearchAnchor should update suggestions on changes to search controller', (WidgetTester tester) async {
final SearchController controller = SearchController();
const List<String> suggestions = <String>['foo','far','bim'];
addTearDown(controller.dispose);
await tester.pumpWidget(MaterialApp(
home: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Material(
child: Align(
alignment: Alignment.topCenter,
child: SearchAnchor(
searchController: controller,
builder: (BuildContext context, SearchController controller) {
return const Icon(Icons.search);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
final String searchText = controller.text.toLowerCase();
if (searchText.isEmpty) {
return const <Widget>[
Center(
child: Text('No Search'),
),
];
}
final Iterable<String> filterSuggestions = suggestions.where(
(String suggestion) => suggestion.toLowerCase().contains(searchText),
);
return filterSuggestions.map((String suggestion) {
return ListTile(
title: Text(suggestion),
trailing: IconButton(
icon: const Icon(Icons.call_missed),
onPressed: () {
controller.text = suggestion;
},
),
onTap: () {
controller.closeView(suggestion);
},
);
}).toList();
},
),
),
);
}
),
));
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
final Finder listTile1 = find.widgetWithText(ListTile, 'foo');
final Finder listTile2 = find.widgetWithText(ListTile, 'far');
final Finder listTile3 = find.widgetWithText(ListTile, 'bim');
final Finder textWidget = find.widgetWithText(Center, 'No Search');
final Finder iconInListTile1 = find.descendant(of: listTile1, matching: find.byIcon(Icons.call_missed));
expect(textWidget,findsOneWidget);
expect(listTile1, findsNothing);
expect(listTile2, findsNothing);
expect(listTile3, findsNothing);
await tester.enterText(find.byType(SearchBar), 'f');
await tester.pumpAndSettle();
expect(textWidget,findsNothing);
expect(listTile1, findsOneWidget);
expect(listTile2, findsOneWidget);
expect(listTile3, findsNothing);
await tester.tap(iconInListTile1);
await tester.pumpAndSettle();
expect(controller.value.text, 'foo');
expect(textWidget,findsNothing);
expect(listTile1, findsOneWidget);
expect(listTile2, findsNothing);
expect(listTile3, findsNothing);
await tester.tap(listTile1);
await tester.pumpAndSettle();
expect(controller.isOpen, false);
expect(controller.value.text, 'foo');
expect(textWidget,findsNothing);
expect(listTile1, findsNothing);
expect(listTile2, findsNothing);
expect(listTile3, findsNothing);
});
testWidgets('SearchAnchor suggestionsBuilder property could be async', (WidgetTester tester) async {
final SearchController controller = SearchController();
addTearDown(controller.dispose);
const String suggestion = 'suggestion text';
await tester.pumpWidget(MaterialApp(
home: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
return Material(
child: Align(
alignment: Alignment.topCenter,
child: SearchAnchor(
searchController: controller,
builder: (BuildContext context, SearchController controller) {
return const Icon(Icons.search);
},
suggestionsBuilder: (BuildContext context, SearchController controller) async {
return <Widget>[
ListTile(
title: const Text(suggestion),
onTap: () {
setState(() {
controller.closeView(suggestion);
});
},
),
];
},
),
),
);
},
),
));
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
final Finder text = find.text(suggestion);
expect(text, findsOneWidget);
await tester.tap(text);
await tester.pumpAndSettle();
expect(controller.isOpen, false);
expect(controller.value.text, suggestion);
});
testWidgets('SearchAnchor.bar has a default search bar as the anchor', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Material(
child: Align(
alignment: Alignment.topLeft,
child: SearchAnchor.bar(
isFullScreen: false,
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),),
);
expect(find.byType(SearchBar), findsOneWidget);
final Rect anchorRect = tester.getRect(find.byType(SearchBar));
expect(anchorRect.size, const Size(800.0, 56.0));
expect(anchorRect, equals(const Rect.fromLTRB(0.0, 0.0, 800.0, 56.0)));
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
final Rect searchViewRect = tester.getRect(find.descendant(of: findViewContent(), matching: find.byType(SizedBox)).first);
expect(searchViewRect, equals(const Rect.fromLTRB(0.0, 0.0, 800.0, 400.0)));
// Search view has same width with the default anchor(search bar).
expect(searchViewRect.width, anchorRect.width);
});
testWidgets('SearchController can open/close view', (WidgetTester tester) async {
final SearchController controller = SearchController();
addTearDown(controller.dispose);
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SearchAnchor.bar(
searchController: controller,
isFullScreen: false,
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[
ListTile(
title: const Text('item 0'),
onTap: () {
controller.closeView('item 0');
},
)
];
},
),
),
),
);
expect(controller.isOpen, false);
await tester.tap(find.byType(SearchBar));
await tester.pumpAndSettle();
expect(controller.isOpen, true);
await tester.tap(find.widgetWithText(ListTile, 'item 0'));
await tester.pumpAndSettle();
expect(controller.isOpen, false);
controller.openView();
expect(controller.isOpen, true);
});
testWidgets('Search view does not go off the screen - LTR', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Align(
// Put the search anchor on the bottom-right corner of the screen to test
// if the search view goes off the window.
alignment: Alignment.bottomRight,
child: SearchAnchor(
isFullScreen: false,
builder: (BuildContext context, SearchController controller) {
return IconButton(
icon: const Icon(Icons.search),
onPressed: () {
controller.openView();
},
);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
),
);
final Finder findIconButton = find.widgetWithIcon(IconButton, Icons.search);
final Rect iconButton = tester.getRect(findIconButton);
// Icon button has a size of (48.0, 48.0) and the screen size is (800.0, 600.0).
expect(iconButton, equals(const Rect.fromLTRB(752.0, 552.0, 800.0, 600.0)));
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
final Rect searchViewRect = tester.getRect(find.descendant(of: findViewContent(), matching: find.byType(SizedBox)).first);
expect(searchViewRect, equals(const Rect.fromLTRB(440.0, 200.0, 800.0, 600.0)));
});
testWidgets('Search view does not go off the screen - RTL', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Directionality(
textDirection: TextDirection.rtl,
child: Material(
child: Align(
// Put the search anchor on the bottom-left corner of the screen to test
// if the search view goes off the window when the text direction is right-to-left.
alignment: Alignment.bottomLeft,
child: SearchAnchor(
isFullScreen: false,
builder: (BuildContext context, SearchController controller) {
return IconButton(
icon: const Icon(Icons.search),
onPressed: () {
controller.openView();
},
);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
),
),
);
final Finder findIconButton = find.widgetWithIcon(IconButton, Icons.search);
final Rect iconButton = tester.getRect(findIconButton);
expect(iconButton, equals(const Rect.fromLTRB(0.0, 552.0, 48.0, 600.0)));
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
final Rect searchViewRect = tester.getRect(find.descendant(of: findViewContent(), matching: find.byType(SizedBox)).first);
expect(searchViewRect, equals(const Rect.fromLTRB(0.0, 200.0, 360.0, 600.0)));
});
testWidgets('Search view becomes smaller if the window size is smaller than the view size', (WidgetTester tester) async {
addTearDown(tester.view.reset);
tester.view.physicalSize = const Size(200.0, 200.0);
tester.view.devicePixelRatio = 1.0;
Widget buildSearchAnchor({TextDirection textDirection = TextDirection.ltr}) {
return MaterialApp(
home: Directionality(
textDirection: textDirection,
child: Material(
child: SearchAnchor(
isFullScreen: false,
builder: (BuildContext context, SearchController controller) {
return Align(
alignment: Alignment.bottomRight,
child: IconButton(
icon: const Icon(Icons.search),
onPressed: () {
controller.openView();
},
),
);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
);
}
// Test LTR text direction.
await tester.pumpWidget(buildSearchAnchor());
final Finder findIconButton = find.widgetWithIcon(IconButton, Icons.search);
final Rect iconButton = tester.getRect(findIconButton);
// The icon button size is (48.0, 48.0), and the screen size is (200.0, 200.0)
expect(iconButton, equals(const Rect.fromLTRB(152.0, 152.0, 200.0, 200.0)));
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
final Rect searchViewRect = tester.getRect(find.descendant(of: findViewContent(), matching: find.byType(SizedBox)).first);
expect(searchViewRect, equals(const Rect.fromLTRB(0.0, 0.0, 200.0, 200.0)));
// Test RTL text direction.
await tester.pumpWidget(Container());
await tester.pumpWidget(buildSearchAnchor(textDirection: TextDirection.rtl));
final Finder findIconButtonRTL = find.widgetWithIcon(IconButton, Icons.search);
final Rect iconButtonRTL = tester.getRect(findIconButtonRTL);
// The icon button size is (48.0, 48.0), and the screen size is (200.0, 200.0)
expect(iconButtonRTL, equals(const Rect.fromLTRB(152.0, 152.0, 200.0, 200.0)));
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
final Rect searchViewRectRTL = tester.getRect(find.descendant(of: findViewContent(), matching: find.byType(SizedBox)).first);
expect(searchViewRectRTL, equals(const Rect.fromLTRB(0.0, 0.0, 200.0, 200.0)));
});
testWidgets('Docked search view route is popped if the window size changes', (WidgetTester tester) async {
addTearDown(tester.view.reset);
tester.view.physicalSize = const Size(500.0, 600.0);
tester.view.devicePixelRatio = 1.0;
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SearchAnchor(
isFullScreen: false,
builder: (BuildContext context, SearchController controller) {
return Align(
alignment: Alignment.bottomRight,
child: IconButton(
icon: const Icon(Icons.search),
onPressed: () {
controller.openView();
},
),
);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
);
// Open the search view
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
expect(find.byIcon(Icons.arrow_back), findsOneWidget);
// Change window size
tester.view.physicalSize = const Size(250.0, 200.0);
tester.view.devicePixelRatio = 1.0;
await tester.pumpAndSettle();
expect(find.byIcon(Icons.arrow_back), findsNothing);
});
testWidgets('Full-screen search view route should stay if the window size changes', (WidgetTester tester) async {
addTearDown(tester.view.reset);
tester.view.physicalSize = const Size(500.0, 600.0);
tester.view.devicePixelRatio = 1.0;
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SearchAnchor(
isFullScreen: true,
builder: (BuildContext context, SearchController controller) {
return Align(
alignment: Alignment.bottomRight,
child: IconButton(
icon: const Icon(Icons.search),
onPressed: () {
controller.openView();
},
),
);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
);
// Open a full-screen search view
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
expect(find.byIcon(Icons.arrow_back), findsOneWidget);
// Change window size
tester.view.physicalSize = const Size(250.0, 200.0);
tester.view.devicePixelRatio = 1.0;
await tester.pumpAndSettle();
expect(find.byIcon(Icons.arrow_back), findsOneWidget);
});
testWidgets('Search view route does not throw exception during pop animation', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/126590.
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Center(
child: SearchAnchor(
builder: (BuildContext context, SearchController controller) {
return IconButton(
icon: const Icon(Icons.search),
onPressed: () {
controller.openView();
},
);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return List<Widget>.generate(5, (int index) {
final String item = 'item $index';
return ListTile(
leading: const Icon(Icons.history),
title: Text(item),
trailing: const Icon(Icons.chevron_right),
onTap: () {},
);
});
}),
),
),
),
);
// Open search view
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
// Pop search view route
await tester.tap(find.byIcon(Icons.arrow_back));
await tester.pumpAndSettle();
// No exception.
});
testWidgets('Docked search should position itself correctly based on closest navigator', (WidgetTester tester) async {
const double rootSpacing = 100.0;
await tester.pumpWidget(
MaterialApp(
builder: (BuildContext context, Widget? child) {
return Scaffold(
body: Padding(
padding: const EdgeInsets.all(rootSpacing),
child: child,
),
);
},
home: Material(
child: SearchAnchor(
isFullScreen: false,
builder: (BuildContext context, SearchController controller) {
return IconButton(
icon: const Icon(Icons.search),
onPressed: () {
controller.openView();
},
);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
);
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
final Rect searchViewRect = tester.getRect(find.descendant(of: findViewContent(), matching: find.byType(SizedBox)).first);
expect(searchViewRect.topLeft, equals(const Offset(rootSpacing, rootSpacing)));
});
testWidgets('Docked search view with nested navigator does not go off the screen', (WidgetTester tester) async {
addTearDown(tester.view.reset);
tester.view.physicalSize = const Size(400.0, 400.0);
tester.view.devicePixelRatio = 1.0;
const double rootSpacing = 100.0;
await tester.pumpWidget(
MaterialApp(
builder: (BuildContext context, Widget? child) {
return Scaffold(
body: Padding(
padding: const EdgeInsets.all(rootSpacing),
child: child,
),
);
},
home: Material(
child: Align(
alignment: Alignment.bottomRight,
child: SearchAnchor(
isFullScreen: false,
builder: (BuildContext context, SearchController controller) {
return IconButton(
icon: const Icon(Icons.search),
onPressed: () {
controller.openView();
},
);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
),
);
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
final Rect searchViewRect = tester.getRect(find.descendant(of: findViewContent(), matching: find.byType(SizedBox)).first);
expect(searchViewRect.bottomRight, equals(const Offset(300.0, 300.0)));
});
// Regression tests for https://github.com/flutter/flutter/issues/128332
group('SearchAnchor text selection', () {
testWidgets('can right-click to select word', (WidgetTester tester) async {
const String defaultText = 'initial text';
final SearchController controller = SearchController();
addTearDown(controller.dispose);
controller.text = defaultText;
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SearchAnchor.bar(
searchController: controller,
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
);
expect(controller.value.text, defaultText);
expect(find.text(defaultText), findsOneWidget);
final TestGesture gesture = await tester.startGesture(
textOffsetToPosition(tester, 4) + const Offset(0.0, -9.0),
kind: PointerDeviceKind.mouse,
buttons: kSecondaryMouseButton,
);
await tester.pump();
await gesture.up();
await tester.pumpAndSettle();
expect(controller.value.selection, const TextSelection(baseOffset: 0, extentOffset: 7));
await gesture.removePointer();
}, variant: TargetPlatformVariant.only(TargetPlatform.macOS));
testWidgets('can click to set position', (WidgetTester tester) async {
const String defaultText = 'initial text';
final SearchController controller = SearchController();
addTearDown(controller.dispose);
controller.text = defaultText;
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SearchAnchor.bar(
searchController: controller,
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
);
expect(controller.value.text, defaultText);
expect(find.text(defaultText), findsOneWidget);
final TestGesture gesture = await _pointGestureToSearchBar(tester);
await gesture.down(textOffsetToPosition(tester, 2) + const Offset(0.0, -9.0));
await tester.pump();
await gesture.up();
await tester.pumpAndSettle(kDoubleTapTimeout);
expect(controller.value.selection, const TextSelection.collapsed(offset: 2));
await gesture.down(textOffsetToPosition(tester, 9, index: 1) + const Offset(0.0, -9.0));
await tester.pump();
await gesture.up();
await tester.pumpAndSettle();
expect(controller.value.selection, const TextSelection.collapsed(offset: 9));
await gesture.removePointer();
}, variant: TargetPlatformVariant.desktop());
testWidgets('can double-click to select word', (WidgetTester tester) async {
const String defaultText = 'initial text';
final SearchController controller = SearchController();
addTearDown(controller.dispose);
controller.text = defaultText;
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SearchAnchor.bar(
searchController: controller,
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
);
expect(controller.value.text, defaultText);
expect(find.text(defaultText), findsOneWidget);
final TestGesture gesture = await _pointGestureToSearchBar(tester);
final Offset targetPosition = textOffsetToPosition(tester, 4) + const Offset(0.0, -9.0);
await gesture.down(targetPosition);
await tester.pump();
await gesture.up();
await tester.pumpAndSettle(kDoubleTapTimeout);
final Offset targetPositionAfterViewOpened = textOffsetToPosition(tester, 4, index: 1) + const Offset(0.0, -9.0);
await gesture.down(targetPositionAfterViewOpened);
await tester.pumpAndSettle();
await gesture.up();
await tester.pump();
await gesture.down(targetPositionAfterViewOpened);
await tester.pump();
await gesture.up();
await tester.pump();
expect(controller.value.selection, const TextSelection(baseOffset: 0, extentOffset: 7));
await gesture.removePointer();
}, variant: TargetPlatformVariant.desktop());
testWidgets('can triple-click to select field', (WidgetTester tester) async {
const String defaultText = 'initial text';
final SearchController controller = SearchController();
addTearDown(controller.dispose);
controller.text = defaultText;
await tester.pumpWidget(
MaterialApp(
home: Material(
child: SearchAnchor.bar(
searchController: controller,
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
);
expect(controller.value.text, defaultText);
expect(find.text(defaultText), findsOneWidget);
final TestGesture gesture = await _pointGestureToSearchBar(tester);
final Offset targetPosition = textOffsetToPosition(tester, 4) + const Offset(0.0, -9.0);
await gesture.down(targetPosition);
await tester.pump();
await gesture.up();
await tester.pumpAndSettle(kDoubleTapTimeout);
final Offset targetPositionAfterViewOpened = textOffsetToPosition(tester, 4, index: 1) + const Offset(0.0, -9.0);
await gesture.down(targetPositionAfterViewOpened);
await tester.pump();
await gesture.up();
await tester.pump();
await gesture.down(targetPositionAfterViewOpened);
await tester.pump();
await gesture.up();
await tester.pump();
await gesture.down(targetPositionAfterViewOpened);
await tester.pump();
await gesture.up();
await tester.pumpAndSettle();
expect(controller.value.selection, const TextSelection(baseOffset: 0, extentOffset: 12));
await gesture.removePointer();
}, variant: TargetPlatformVariant.desktop());
});
// Regression tests for https://github.com/flutter/flutter/issues/126623
group('Overall InputDecorationTheme does not impact SearchBar and SearchView', () {
const InputDecorationTheme inputDecorationTheme = InputDecorationTheme(
focusColor: Colors.green,
hoverColor: Colors.blue,
outlineBorder: BorderSide(color: Colors.pink, width: 10),
isDense: true,
contentPadding: EdgeInsets.symmetric(horizontal: 20),
hintStyle: TextStyle(color: Colors.purpleAccent),
fillColor: Colors.tealAccent,
filled: true,
isCollapsed: true,
border: OutlineInputBorder(),
focusedBorder: UnderlineInputBorder(),
enabledBorder: UnderlineInputBorder(),
errorBorder: UnderlineInputBorder(),
focusedErrorBorder: UnderlineInputBorder(),
disabledBorder: UnderlineInputBorder(),
constraints: BoxConstraints(maxWidth: 300),
);
final ThemeData theme = ThemeData(
useMaterial3: true,
inputDecorationTheme: inputDecorationTheme
);
void checkDecorationInSearchBar(WidgetTester tester) {
final Finder textField = findTextField();
final InputDecoration? decoration = tester.widget<TextField>(textField).decoration;
expect(decoration?.border, InputBorder.none);
expect(decoration?.focusedBorder, InputBorder.none);
expect(decoration?.enabledBorder, InputBorder.none);
expect(decoration?.errorBorder, null);
expect(decoration?.focusedErrorBorder, null);
expect(decoration?.disabledBorder, null);
expect(decoration?.constraints, null);
expect(decoration?.isCollapsed, false);
expect(decoration?.filled, false);
expect(decoration?.fillColor, null);
expect(decoration?.focusColor, null);
expect(decoration?.hoverColor, null);
expect(decoration?.contentPadding, EdgeInsets.zero);
expect(decoration?.hintStyle?.color, theme.colorScheme.onSurfaceVariant);
}
testWidgets('Overall InputDecorationTheme does not override text field style'
' in SearchBar', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: theme,
home: const Center(
child: Material(
child: SearchBar(hintText: 'hint text'),
),
),
),
);
// Check input decoration in `SearchBar`
checkDecorationInSearchBar(tester);
// Check search bar defaults.
final Finder searchBarMaterial = find.descendant(
of: find.byType(SearchBar),
matching: find.byType(Material),
);
final Material material = tester.widget<Material>(searchBarMaterial);
checkSearchBarDefaults(tester, theme.colorScheme, material);
});
testWidgets('Overall InputDecorationTheme does not override text field style'
' in the search view route', (WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
theme: theme,
home: Scaffold(
body: Material(
child: Align(
alignment: Alignment.topLeft,
child: SearchAnchor(
viewHintText: 'hint text',
builder: (BuildContext context, SearchController controller) {
return const Icon(Icons.search);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
),
),
);
await tester.tap(find.byIcon(Icons.search));
await tester.pumpAndSettle();
// Check input decoration in `SearchBar`
checkDecorationInSearchBar(tester);
// Check search bar defaults in search view route.
final Finder searchBarMaterial = find.descendant(
of: find.descendant(of: findViewContent(), matching: find.byType(SearchBar)),
matching: find.byType(Material),
).first;
final Material material = tester.widget<Material>(searchBarMaterial);
expect(material.color, Colors.transparent);
expect(material.elevation, 0.0);
final Text hintText = tester.widget(find.text('hint text'));
expect(hintText.style?.color, theme.colorScheme.onSurfaceVariant);
const String input = 'entered text';
await tester.enterText(find.byType(SearchBar), input);
final EditableText inputText = tester.widget(find.text(input));
expect(inputText.style.color, theme.colorScheme.onSurface);
});
});
testWidgets('SearchAnchor view respects theme brightness', (WidgetTester tester) async {
Widget buildSearchAnchor(ThemeData theme) {
return MaterialApp(
theme: theme,
home: Center(
child: Material(
child: SearchAnchor(
builder: (BuildContext context, SearchController controller) {
return IconButton(
icon: const Icon(Icons.ac_unit),
onPressed: () {
controller.openView();
},
);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
);
}
ThemeData theme = ThemeData(brightness: Brightness.light);
await tester.pumpWidget(buildSearchAnchor(theme));
// Open the search view.
await tester.tap(find.widgetWithIcon(IconButton, Icons.ac_unit));
await tester.pumpAndSettle();
// Test the search view background color.
Material material = getSearchViewMaterial(tester);
expect(material.color, theme.colorScheme.surfaceContainerHigh);
// Change the theme brightness.
theme = ThemeData(brightness: Brightness.dark);
await tester.pumpWidget(buildSearchAnchor(theme));
await tester.pumpAndSettle();
// Test the search view background color.
material = getSearchViewMaterial(tester);
expect(material.color, theme.colorScheme.surfaceContainerHigh);
});
testWidgets('Search view widgets can inherit local themes', (WidgetTester tester) async {
final ThemeData globalTheme = ThemeData(colorSchemeSeed: Colors.red);
final ThemeData localTheme = ThemeData(
colorSchemeSeed: Colors.green,
iconButtonTheme: IconButtonThemeData(
style: IconButton.styleFrom(
backgroundColor: const Color(0xffffff00)
),
),
cardTheme: const CardTheme(color: Color(0xff00ffff)),
);
Widget buildSearchAnchor() {
return MaterialApp(
theme: globalTheme,
home: Center(
child: Builder(
builder: (BuildContext context) {
return Theme(
data: localTheme,
child: Material(
child: SearchAnchor.bar(
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[
Card(
child: ListTile(
onTap: () {},
title: const Text('Item 1'),
),
),
];
},
),
),
);
}
),
),
);
}
await tester.pumpWidget(buildSearchAnchor());
// Open the search view.
await tester.tap(find.byType(SearchBar));
await tester.pumpAndSettle();
// Test the search view background color.
final Material searchViewMaterial = getSearchViewMaterial(tester);
expect(searchViewMaterial.color, localTheme.colorScheme.surfaceContainerHigh);
// Test the search view icons background color.
final Material iconButtonMaterial = tester.widget<Material>(find.descendant(
of: find.byType(IconButton),
matching: find.byType(Material),
).first);
expect(find.byWidget(iconButtonMaterial), findsOneWidget);
expect(iconButtonMaterial.color, localTheme.iconButtonTheme.style?.backgroundColor?.resolve(<MaterialState>{}));
// Test the suggestion card color.
final Material suggestionMaterial = tester.widget<Material>(find.descendant(
of: find.byType(Card),
matching: find.byType(Material),
).first);
expect(suggestionMaterial.color, localTheme.cardTheme.color);
});
testWidgets('SearchBar respects keyboardType property', (WidgetTester tester) async {
Widget buildSearchBar(TextInputType keyboardType) {
return MaterialApp(
home: Center(
child: Material(
child: SearchBar(
keyboardType: keyboardType,
),
),
),
);
}
await tester.pumpWidget(buildSearchBar(TextInputType.number));
await tester.pump();
TextField textField = tester.widget(find.byType(TextField));
expect(textField.keyboardType, TextInputType.number);
await tester.pumpWidget(buildSearchBar(TextInputType.phone));
await tester.pump();
textField = tester.widget(find.byType(TextField));
expect(textField.keyboardType, TextInputType.phone);
});
testWidgets('SearchAnchor respects keyboardType property', (WidgetTester tester) async {
Widget buildSearchAnchor(TextInputType keyboardType) {
return MaterialApp(
home: Center(
child: Material(
child: SearchAnchor(
keyboardType: keyboardType,
builder: (BuildContext context, SearchController controller) {
return IconButton(
icon: const Icon(Icons.ac_unit),
onPressed: () {
controller.openView();
},
);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
);
}
await tester.pumpWidget(buildSearchAnchor(TextInputType.number));
await tester.pump();
await tester.tap(find.widgetWithIcon(IconButton, Icons.ac_unit));
await tester.pumpAndSettle();
TextField textField = tester.widget(find.byType(TextField));
expect(textField.keyboardType, TextInputType.number);
await tester.tap(find.widgetWithIcon(IconButton, Icons.arrow_back));
await tester.pump();
await tester.pumpWidget(buildSearchAnchor(TextInputType.phone));
await tester.pump();
await tester.tap(find.widgetWithIcon(IconButton, Icons.ac_unit));
await tester.pumpAndSettle();
textField = tester.widget(find.byType(TextField));
expect(textField.keyboardType, TextInputType.phone);
});
testWidgets('SearchAnchor.bar respects keyboardType property', (WidgetTester tester) async {
Widget buildSearchAnchor(TextInputType keyboardType) {
return MaterialApp(
home: Center(
child: Material(
child: SearchAnchor.bar(
keyboardType: keyboardType,
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
);
}
await tester.pumpWidget(buildSearchAnchor(TextInputType.number));
await tester.pump();
await tester.tap(find.byType(SearchBar)); // Open search view.
await tester.pumpAndSettle();
final Finder textFieldFinder = find.descendant(of: findViewContent(), matching: find.byType(TextField));
final TextField textFieldInView = tester.widget<TextField>(textFieldFinder);
expect(textFieldInView.keyboardType, TextInputType.number);
// Close search view.
await tester.tap(find.widgetWithIcon(IconButton, Icons.arrow_back));
await tester.pumpAndSettle();
final TextField textField = tester.widget(find.byType(TextField));
expect(textField.keyboardType, TextInputType.number);
});
testWidgets('SearchBar respects textInputAction property', (WidgetTester tester) async {
Widget buildSearchBar(TextInputAction textInputAction) {
return MaterialApp(
home: Center(
child: Material(
child: SearchBar(
textInputAction: textInputAction,
),
),
),
);
}
await tester.pumpWidget(buildSearchBar(TextInputAction.previous));
await tester.pump();
TextField textField = tester.widget(find.byType(TextField));
expect(textField.textInputAction, TextInputAction.previous);
await tester.pumpWidget(buildSearchBar(TextInputAction.send));
await tester.pump();
textField = tester.widget(find.byType(TextField));
expect(textField.textInputAction, TextInputAction.send);
});
testWidgets('SearchAnchor respects textInputAction property', (WidgetTester tester) async {
Widget buildSearchAnchor(TextInputAction textInputAction) {
return MaterialApp(
home: Center(
child: Material(
child: SearchAnchor(
textInputAction: textInputAction,
builder: (BuildContext context, SearchController controller) {
return IconButton(
icon: const Icon(Icons.ac_unit),
onPressed: () {
controller.openView();
},
);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
);
}
await tester.pumpWidget(buildSearchAnchor(TextInputAction.previous));
await tester.pump();
await tester.tap(find.widgetWithIcon(IconButton, Icons.ac_unit));
await tester.pumpAndSettle();
TextField textField = tester.widget(find.byType(TextField));
expect(textField.textInputAction, TextInputAction.previous);
await tester.tap(find.widgetWithIcon(IconButton, Icons.arrow_back));
await tester.pump();
await tester.pumpWidget(buildSearchAnchor(TextInputAction.send));
await tester.pump();
await tester.tap(find.widgetWithIcon(IconButton, Icons.ac_unit));
await tester.pumpAndSettle();
textField = tester.widget(find.byType(TextField));
expect(textField.textInputAction, TextInputAction.send);
});
testWidgets('SearchAnchor.bar respects textInputAction property', (WidgetTester tester) async {
Widget buildSearchAnchor(TextInputAction textInputAction) {
return MaterialApp(
home: Center(
child: Material(
child: SearchAnchor.bar(
textInputAction: textInputAction,
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
);
}
await tester.pumpWidget(buildSearchAnchor(TextInputAction.previous));
await tester.pump();
await tester.tap(find.byType(SearchBar)); // Open search view.
await tester.pumpAndSettle();
final Finder textFieldFinder = find.descendant(of: findViewContent(), matching: find.byType(TextField));
final TextField textFieldInView = tester.widget<TextField>(textFieldFinder);
expect(textFieldInView.textInputAction, TextInputAction.previous);
// Close search view.
await tester.tap(find.widgetWithIcon(IconButton, Icons.arrow_back));
await tester.pumpAndSettle();
final TextField textField = tester.widget(find.byType(TextField));
expect(textField.textInputAction, TextInputAction.previous);
});
testWidgets('Block entering text on disabled widget', (WidgetTester tester) async {
const String initValue = 'init';
final TextEditingController controller = TextEditingController(text: initValue);
addTearDown(controller.dispose);
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Center(
child: SearchBar(
controller: controller,
enabled: false,
),
),
),
),
);
const String testValue = 'abcdefghi';
await tester.enterText(find.byType(SearchBar), testValue);
expect(controller.value.text, initValue);
});
testWidgets('Disabled SearchBar semantics node still contains value', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
final TextEditingController controller = TextEditingController(text: 'text');
addTearDown(controller.dispose);
await tester.pumpWidget(
MaterialApp(
home: Material(
child: Center(
child: SearchBar(
controller: controller,
enabled: false,
),
),
),
),
);
expect(semantics, includesNodeWith(actions: <SemanticsAction>[], value: 'text'));
semantics.dispose();
});
testWidgets('Check SearchBar opacity when disabled', (WidgetTester tester) async {
await tester.pumpWidget(
const MaterialApp(
home: Material(
child: Center(
child: SearchBar(
enabled: false,
),
),
),
),
);
final Finder searchBarFinder = find.byType(SearchBar);
expect(searchBarFinder, findsOneWidget);
final Finder opacityFinder = find.descendant(
of: searchBarFinder,
matching: find.byType(Opacity),
);
expect(opacityFinder, findsOneWidget);
final Opacity opacityWidget = tester.widget<Opacity>(opacityFinder);
expect(opacityWidget.opacity, 0.38);
});
testWidgets('SearchAnchor respects headerHeight', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Center(
child: Material(
child: SearchAnchor(
isFullScreen: true,
builder: (BuildContext context, SearchController controller) {
return const Icon(Icons.search);
},
headerHeight: 32,
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
));
await tester.pump();
await tester.tap(find.byIcon(Icons.search)); // Open search view.
await tester.pumpAndSettle();
final Finder findHeader = find.descendant(of: findViewContent(), matching: find.byType(SearchBar));
expect(tester.getSize(findHeader).height, 32);
});
testWidgets('SearchAnchor.bar respects viewHeaderHeight', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Center(
child: Material(
child: SearchAnchor.bar(
isFullScreen: true,
viewHeaderHeight: 32,
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
));
await tester.pump();
await tester.tap(find.byType(SearchBar)); // Open search view.
await tester.pumpAndSettle();
final Finder findHeader = find.descendant(of: findViewContent(), matching: find.byType(SearchBar));
final RenderBox box = tester.renderObject(findHeader);
expect(box.size.height, 32);
});
testWidgets('The default clear button only shows when text input is not empty '
'on the search view', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Center(
child: Material(
child: SearchAnchor(
builder: (BuildContext context, SearchController controller) {
return const Icon(Icons.search);
},
suggestionsBuilder: (BuildContext context, SearchController controller) {
return <Widget>[];
},
),
),
),
));
await tester.pump();
await tester.tap(find.byIcon(Icons.search)); // Open search view.
await tester.pumpAndSettle();
expect(find.widgetWithIcon(IconButton, Icons.close), findsNothing);
await tester.enterText(findTextField(), 'a');
await tester.pump();
expect(find.widgetWithIcon(IconButton, Icons.close), findsOneWidget);
await tester.enterText(findTextField(), '');
await tester.pump();
expect(find.widgetWithIcon(IconButton, Icons.close), findsNothing);
});
}
Future<void> checkSearchBarDefaults(WidgetTester tester, ColorScheme colorScheme, Material material) async {
expect(material.animationDuration, const Duration(milliseconds: 200));
expect(material.borderOnForeground, true);
expect(material.borderRadius, null);
expect(material.clipBehavior, Clip.none);
expect(material.color, colorScheme.surfaceContainerHigh);
expect(material.elevation, 6.0);
expect(material.shadowColor, colorScheme.shadow);
expect(material.surfaceTintColor, Colors.transparent);
expect(material.shape, const StadiumBorder());
final Text helperText = tester.widget(find.text('hint text'));
expect(helperText.style?.color, colorScheme.onSurfaceVariant);
expect(helperText.style?.fontSize, 16.0);
expect(helperText.style?.fontFamily, 'Roboto');
expect(helperText.style?.fontWeight, FontWeight.w400);
const String input = 'entered text';
await tester.enterText(find.byType(SearchBar), input);
final EditableText inputText = tester.widget(find.text(input));
expect(inputText.style.color, colorScheme.onSurface);
expect(inputText.style.fontSize, 16.0);
expect(helperText.style?.fontFamily, 'Roboto');
expect(inputText.style.fontWeight, FontWeight.w400);
}
Finder findTextField() {
return find.descendant(
of: find.byType(SearchBar),
matching: find.byType(TextField)
);
}
TextStyle? _iconStyle(WidgetTester tester, IconData icon) {
final RichText iconRichText = tester.widget<RichText>(
find.descendant(of: find.byIcon(icon), matching: find.byType(RichText)),
);
return iconRichText.text.style;
}
const Color pressedColor = Colors.red;
const Color hoveredColor = Colors.orange;
const Color focusedColor = Colors.yellow;
const Color defaultColor = Colors.green;
Color _getColor(Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return pressedColor;
}
if (states.contains(MaterialState.hovered)) {
return hoveredColor;
}
if (states.contains(MaterialState.focused)) {
return focusedColor;
}
return defaultColor;
}
final ThemeData theme = ThemeData();
final TextStyle? pressedStyle = theme.textTheme.bodyLarge?.copyWith(color: pressedColor);
final TextStyle? hoveredStyle = theme.textTheme.bodyLarge?.copyWith(color: hoveredColor);
final TextStyle? focusedStyle = theme.textTheme.bodyLarge?.copyWith(color: focusedColor);
TextStyle? _getTextStyle(Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
return pressedStyle;
}
if (states.contains(MaterialState.hovered)) {
return hoveredStyle;
}
if (states.contains(MaterialState.focused)) {
return focusedStyle;
}
return null;
}
Future<TestGesture> _pointGestureToSearchBar(WidgetTester tester) async {
final Offset center = tester.getCenter(find.byType(SearchBar));
final TestGesture gesture = await tester.createGesture(
kind: PointerDeviceKind.mouse,
);
// On hovered.
await gesture.addPointer();
await gesture.moveTo(center);
return gesture;
}
Finder findViewContent() {
return find.byWidgetPredicate((Widget widget) {
return widget.runtimeType.toString() == '_ViewContent';
});
}
Material getSearchViewMaterial(WidgetTester tester) {
return tester.widget<Material>(find.descendant(of: findViewContent(), matching: find.byType(Material)).first);
}
| flutter/packages/flutter/test/material/search_anchor_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/search_anchor_test.dart",
"repo_id": "flutter",
"token_count": 46619
} | 694 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('SwitchThemeData copyWith, ==, hashCode basics', () {
expect(const SwitchThemeData(), const SwitchThemeData().copyWith());
expect(const SwitchThemeData().hashCode, const SwitchThemeData().copyWith().hashCode);
});
test('SwitchThemeData lerp special cases', () {
const SwitchThemeData data = SwitchThemeData();
expect(identical(SwitchThemeData.lerp(data, data, 0.5), data), true);
});
test('SwitchThemeData defaults', () {
const SwitchThemeData themeData = SwitchThemeData();
expect(themeData.thumbColor, null);
expect(themeData.trackColor, null);
expect(themeData.trackOutlineColor, null);
expect(themeData.trackOutlineWidth, null);
expect(themeData.mouseCursor, null);
expect(themeData.materialTapTargetSize, null);
expect(themeData.overlayColor, null);
expect(themeData.splashRadius, null);
expect(themeData.thumbIcon, null);
const SwitchTheme theme = SwitchTheme(data: SwitchThemeData(), child: SizedBox());
expect(theme.data.thumbColor, null);
expect(theme.data.trackColor, null);
expect(theme.data.trackOutlineColor, null);
expect(theme.data.trackOutlineWidth, null);
expect(theme.data.mouseCursor, null);
expect(theme.data.materialTapTargetSize, null);
expect(theme.data.overlayColor, null);
expect(theme.data.splashRadius, null);
expect(theme.data.thumbIcon, null);
});
testWidgets('Default SwitchThemeData debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const SwitchThemeData().debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description, <String>[]);
});
testWidgets('SwitchThemeData implements debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const SwitchThemeData(
thumbColor: MaterialStatePropertyAll<Color>(Color(0xfffffff0)),
trackColor: MaterialStatePropertyAll<Color>(Color(0xfffffff1)),
trackOutlineColor: MaterialStatePropertyAll<Color>(Color(0xfffffff3)),
trackOutlineWidth: MaterialStatePropertyAll<double>(6.0),
mouseCursor: MaterialStatePropertyAll<MouseCursor>(SystemMouseCursors.click),
materialTapTargetSize: MaterialTapTargetSize.shrinkWrap,
overlayColor: MaterialStatePropertyAll<Color>(Color(0xfffffff2)),
splashRadius: 1.0,
thumbIcon: MaterialStatePropertyAll<Icon>(Icon(IconData(123))),
).debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description[0], 'thumbColor: WidgetStatePropertyAll(Color(0xfffffff0))');
expect(description[1], 'trackColor: WidgetStatePropertyAll(Color(0xfffffff1))');
expect(description[2], 'trackOutlineColor: WidgetStatePropertyAll(Color(0xfffffff3))');
expect(description[3], 'trackOutlineWidth: WidgetStatePropertyAll(6.0)');
expect(description[4], 'materialTapTargetSize: MaterialTapTargetSize.shrinkWrap');
expect(description[5], 'mouseCursor: WidgetStatePropertyAll(SystemMouseCursor(click))');
expect(description[6], 'overlayColor: WidgetStatePropertyAll(Color(0xfffffff2))');
expect(description[7], 'splashRadius: 1.0');
expect(description[8], 'thumbIcon: WidgetStatePropertyAll(Icon(IconData(U+0007B)))');
});
testWidgets('Material2 - Switch is themeable', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
const Color defaultThumbColor = Color(0xfffffff0);
const Color selectedThumbColor = Color(0xfffffff1);
const Color defaultTrackColor = Color(0xfffffff2);
const Color selectedTrackColor = Color(0xfffffff3);
const Color defaultTrackOutlineColor = Color(0xfffffff4);
const Color selectedTrackOutlineColor = Color(0xfffffff5);
const double defaultTrackOutlineWidth = 3.0;
const double selectedTrackOutlineWidth = 6.0;
const MouseCursor mouseCursor = SystemMouseCursors.text;
const MaterialTapTargetSize materialTapTargetSize = MaterialTapTargetSize.shrinkWrap;
const Color focusOverlayColor = Color(0xfffffff4);
const Color hoverOverlayColor = Color(0xfffffff5);
const double splashRadius = 1.0;
const Icon icon1 = Icon(Icons.check);
const Icon icon2 = Icon(Icons.close);
final ThemeData themeData = ThemeData(
useMaterial3: false,
switchTheme: SwitchThemeData(
thumbColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return selectedThumbColor;
}
return defaultThumbColor;
}),
trackColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return selectedTrackColor;
}
return defaultTrackColor;
}),
trackOutlineColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return selectedTrackOutlineColor;
}
return defaultTrackOutlineColor;
}),
trackOutlineWidth: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return selectedTrackOutlineWidth;
}
return defaultTrackOutlineWidth;
}),
mouseCursor: const MaterialStatePropertyAll<MouseCursor>(mouseCursor),
materialTapTargetSize: materialTapTargetSize,
overlayColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.focused)) {
return focusOverlayColor;
}
if (states.contains(MaterialState.hovered)) {
return hoverOverlayColor;
}
return null;
}),
splashRadius: splashRadius,
thumbIcon: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return icon1;
}
return icon2;
}),
),
);
Widget buildSwitch({bool selected = false, bool autofocus = false}) {
return MaterialApp(
theme: themeData,
home: Scaffold(
body: Switch(
dragStartBehavior: DragStartBehavior.down,
value: selected,
onChanged: (bool value) {},
autofocus: autofocus,
),
),
);
}
// Switch.
await tester.pumpWidget(buildSwitch());
await tester.pumpAndSettle();
expect(
_getSwitchMaterial(tester),
paints
..rrect(color: defaultTrackColor)
..rrect(color: defaultTrackOutlineColor, strokeWidth: defaultTrackOutlineWidth)
..rrect()
..rrect()
..rrect()
..rrect(color: defaultThumbColor)
);
// Size from MaterialTapTargetSize.shrinkWrap.
expect(tester.getSize(find.byType(Switch)), const Size(59.0, 40.0));
// Selected switch.
await tester.pumpWidget(buildSwitch(selected: true));
await tester.pumpAndSettle();
expect(
_getSwitchMaterial(tester),
paints
..rrect(color: selectedTrackColor)
..rrect(color: selectedTrackOutlineColor, strokeWidth: selectedTrackOutlineWidth)
..rrect()
..rrect()
..rrect()
..rrect(color: selectedThumbColor)
);
// Switch with hover.
await tester.pumpWidget(buildSwitch());
await _pointGestureToSwitch(tester);
await tester.pumpAndSettle();
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.text);
expect(_getSwitchMaterial(tester), paints..circle(color: hoverOverlayColor));
// Switch with focus.
await tester.pumpWidget(buildSwitch(autofocus: true));
await tester.pumpAndSettle();
expect(_getSwitchMaterial(tester), paints..circle(color: focusOverlayColor, radius: splashRadius));
});
testWidgets('Material3 - Switch is themeable', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
const Color defaultThumbColor = Color(0xfffffff0);
const Color selectedThumbColor = Color(0xfffffff1);
const Color defaultTrackColor = Color(0xfffffff2);
const Color selectedTrackColor = Color(0xfffffff3);
const Color defaultTrackOutlineColor = Color(0xfffffff4);
const Color selectedTrackOutlineColor = Color(0xfffffff5);
const double defaultTrackOutlineWidth = 3.0;
const double selectedTrackOutlineWidth = 6.0;
const MouseCursor mouseCursor = SystemMouseCursors.text;
const MaterialTapTargetSize materialTapTargetSize = MaterialTapTargetSize.shrinkWrap;
const Color focusOverlayColor = Color(0xfffffff4);
const Color hoverOverlayColor = Color(0xfffffff5);
const double splashRadius = 1.0;
const Icon icon1 = Icon(Icons.check);
const Icon icon2 = Icon(Icons.close);
final ThemeData themeData = ThemeData(
useMaterial3: true,
switchTheme: SwitchThemeData(
thumbColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return selectedThumbColor;
}
return defaultThumbColor;
}),
trackColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return selectedTrackColor;
}
return defaultTrackColor;
}),
trackOutlineColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return selectedTrackOutlineColor;
}
return defaultTrackOutlineColor;
}),
trackOutlineWidth: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return selectedTrackOutlineWidth;
}
return defaultTrackOutlineWidth;
}),
mouseCursor: const MaterialStatePropertyAll<MouseCursor>(mouseCursor),
materialTapTargetSize: materialTapTargetSize,
overlayColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.focused)) {
return focusOverlayColor;
}
if (states.contains(MaterialState.hovered)) {
return hoverOverlayColor;
}
return null;
}),
splashRadius: splashRadius,
thumbIcon: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return icon1;
}
return icon2;
}),
),
);
Widget buildSwitch({bool selected = false, bool autofocus = false}) {
return MaterialApp(
theme: themeData,
home: Scaffold(
body: Switch(
dragStartBehavior: DragStartBehavior.down,
value: selected,
onChanged: (bool value) {},
autofocus: autofocus,
),
),
);
}
// Switch.
await tester.pumpWidget(buildSwitch());
await tester.pumpAndSettle();
expect(
_getSwitchMaterial(tester),
paints
..rrect(color: defaultTrackColor)
..rrect(color: defaultTrackOutlineColor, strokeWidth: defaultTrackOutlineWidth)
..rrect(color: defaultThumbColor)
..paragraph()
);
// Size from MaterialTapTargetSize.shrinkWrap.
expect(tester.getSize(find.byType(Switch)), const Size(60.0, 40.0));
// Selected switch.
await tester.pumpWidget(buildSwitch(selected: true));
await tester.pumpAndSettle();
expect(
_getSwitchMaterial(tester),
paints
..rrect(color: selectedTrackColor)
..rrect(color: selectedTrackOutlineColor, strokeWidth: selectedTrackOutlineWidth)
..rrect(color: selectedThumbColor)..paragraph()
);
// Switch with hover.
await tester.pumpWidget(buildSwitch());
await _pointGestureToSwitch(tester);
await tester.pumpAndSettle();
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.text);
expect(_getSwitchMaterial(tester), paints..circle(color: hoverOverlayColor));
// Switch with focus.
await tester.pumpWidget(buildSwitch(autofocus: true));
await tester.pumpAndSettle();
expect(_getSwitchMaterial(tester), paints..circle(color: focusOverlayColor, radius: splashRadius));
});
testWidgets('Material2 - Switch properties are taken over the theme values', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
const Color themeDefaultThumbColor = Color(0xfffffff0);
const Color themeSelectedThumbColor = Color(0xfffffff1);
const Color themeDefaultTrackColor = Color(0xfffffff2);
const Color themeSelectedTrackColor = Color(0xfffffff3);
const Color themeDefaultOutlineColor = Color(0xfffffff6);
const Color themeSelectedOutlineColor = Color(0xfffffff7);
const double themeDefaultOutlineWidth = 5.0;
const double themeSelectedOutlineWidth = 7.0;
const MouseCursor themeMouseCursor = SystemMouseCursors.click;
const MaterialTapTargetSize themeMaterialTapTargetSize = MaterialTapTargetSize.padded;
const Color themeFocusOverlayColor = Color(0xfffffff4);
const Color themeHoverOverlayColor = Color(0xfffffff5);
const double themeSplashRadius = 1.0;
const Color defaultThumbColor = Color(0xffffff0f);
const Color selectedThumbColor = Color(0xffffff1f);
const Color defaultTrackColor = Color(0xffffff2f);
const Color selectedTrackColor = Color(0xffffff3f);
const Color defaultOutlineColor = Color(0xffffff6f);
const Color selectedOutlineColor = Color(0xffffff7f);
const double defaultOutlineWidth = 6.0;
const double selectedOutlineWidth = 8.0;
const MouseCursor mouseCursor = SystemMouseCursors.text;
const MaterialTapTargetSize materialTapTargetSize = MaterialTapTargetSize.shrinkWrap;
const Color focusColor = Color(0xffffff4f);
const Color hoverColor = Color(0xffffff5f);
const double splashRadius = 2.0;
final ThemeData themeData = ThemeData(
useMaterial3: false,
switchTheme: SwitchThemeData(
thumbColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return themeSelectedThumbColor;
}
return themeDefaultThumbColor;
}),
trackColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return themeSelectedTrackColor;
}
return themeDefaultTrackColor;
}),
trackOutlineColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return themeSelectedOutlineColor;
}
return themeDefaultOutlineColor;
}),
trackOutlineWidth: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return themeSelectedOutlineWidth;
}
return themeDefaultOutlineWidth;
}),
mouseCursor: const MaterialStatePropertyAll<MouseCursor>(themeMouseCursor),
materialTapTargetSize: themeMaterialTapTargetSize,
overlayColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.focused)) {
return themeFocusOverlayColor;
}
if (states.contains(MaterialState.hovered)) {
return themeHoverOverlayColor;
}
return null;
}),
splashRadius: themeSplashRadius,
thumbIcon: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return null;
}
return null;
}),
),
);
Widget buildSwitch({bool selected = false, bool autofocus = false}) {
return MaterialApp(
theme: themeData,
home: Scaffold(
body: Switch(
value: selected,
onChanged: (bool value) {},
autofocus: autofocus,
thumbColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return selectedThumbColor;
}
return defaultThumbColor;
}),
trackColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return selectedTrackColor;
}
return defaultTrackColor;
}),
trackOutlineColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return selectedOutlineColor;
}
return defaultOutlineColor;
}),
trackOutlineWidth: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return selectedOutlineWidth;
}
return defaultOutlineWidth;
}),
mouseCursor: mouseCursor,
materialTapTargetSize: materialTapTargetSize,
focusColor: focusColor,
hoverColor: hoverColor,
splashRadius: splashRadius,
thumbIcon: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return const Icon(Icons.add);
}
return const Icon(Icons.access_alarm);
}),
),
),
);
}
// Switch.
await tester.pumpWidget(buildSwitch());
await tester.pumpAndSettle();
expect(
_getSwitchMaterial(tester),
paints
..rrect(color: defaultTrackColor)
..rrect(color: defaultOutlineColor, strokeWidth: defaultOutlineWidth)
..rrect()
..rrect()
..rrect()
..rrect(color: defaultThumbColor)
);
// Size from MaterialTapTargetSize.shrinkWrap.
expect(tester.getSize(find.byType(Switch)), const Size(59.0, 40.0));
// Selected switch.
await tester.pumpWidget(buildSwitch(selected: true));
await tester.pumpAndSettle();
expect(
_getSwitchMaterial(tester),
paints
..rrect(color: selectedTrackColor)
..rrect(color: selectedOutlineColor, strokeWidth: selectedOutlineWidth)
..rrect()
..rrect()
..rrect()
..rrect(color: selectedThumbColor)
);
// Switch with hover.
await tester.pumpWidget(buildSwitch());
await _pointGestureToSwitch(tester);
await tester.pumpAndSettle();
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.text);
expect(_getSwitchMaterial(tester), paints..circle(color: hoverColor));
// Switch with focus.
await tester.pumpWidget(buildSwitch(autofocus: true));
await tester.pumpAndSettle();
expect(_getSwitchMaterial(tester), paints..circle(color: focusColor, radius: splashRadius));
});
testWidgets('Material3 - Switch properties are taken over the theme values', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
const Color themeDefaultThumbColor = Color(0xfffffff0);
const Color themeSelectedThumbColor = Color(0xfffffff1);
const Color themeDefaultTrackColor = Color(0xfffffff2);
const Color themeSelectedTrackColor = Color(0xfffffff3);
const Color themeDefaultOutlineColor = Color(0xfffffff6);
const Color themeSelectedOutlineColor = Color(0xfffffff7);
const double themeDefaultOutlineWidth = 5.0;
const double themeSelectedOutlineWidth = 7.0;
const MouseCursor themeMouseCursor = SystemMouseCursors.click;
const MaterialTapTargetSize themeMaterialTapTargetSize = MaterialTapTargetSize.padded;
const Color themeFocusOverlayColor = Color(0xfffffff4);
const Color themeHoverOverlayColor = Color(0xfffffff5);
const double themeSplashRadius = 1.0;
const Color defaultThumbColor = Color(0xffffff0f);
const Color selectedThumbColor = Color(0xffffff1f);
const Color defaultTrackColor = Color(0xffffff2f);
const Color selectedTrackColor = Color(0xffffff3f);
const Color defaultOutlineColor = Color(0xffffff6f);
const Color selectedOutlineColor = Color(0xffffff7f);
const double defaultOutlineWidth = 6.0;
const double selectedOutlineWidth = 8.0;
const MouseCursor mouseCursor = SystemMouseCursors.text;
const MaterialTapTargetSize materialTapTargetSize = MaterialTapTargetSize.shrinkWrap;
const Color focusColor = Color(0xffffff4f);
const Color hoverColor = Color(0xffffff5f);
const double splashRadius = 2.0;
final ThemeData themeData = ThemeData(
useMaterial3: true,
switchTheme: SwitchThemeData(
thumbColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return themeSelectedThumbColor;
}
return themeDefaultThumbColor;
}),
trackColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return themeSelectedTrackColor;
}
return themeDefaultTrackColor;
}),
trackOutlineColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return themeSelectedOutlineColor;
}
return themeDefaultOutlineColor;
}),
trackOutlineWidth: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return themeSelectedOutlineWidth;
}
return themeDefaultOutlineWidth;
}),
mouseCursor: const MaterialStatePropertyAll<MouseCursor>(themeMouseCursor),
materialTapTargetSize: themeMaterialTapTargetSize,
overlayColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.focused)) {
return themeFocusOverlayColor;
}
if (states.contains(MaterialState.hovered)) {
return themeHoverOverlayColor;
}
return null;
}),
splashRadius: themeSplashRadius,
thumbIcon: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return null;
}
return null;
}),
),
);
Widget buildSwitch({bool selected = false, bool autofocus = false}) {
return MaterialApp(
theme: themeData,
home: Scaffold(
body: Switch(
value: selected,
onChanged: (bool value) {},
autofocus: autofocus,
thumbColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return selectedThumbColor;
}
return defaultThumbColor;
}),
trackColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return selectedTrackColor;
}
return defaultTrackColor;
}),
trackOutlineColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return selectedOutlineColor;
}
return defaultOutlineColor;
}),
trackOutlineWidth: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return selectedOutlineWidth;
}
return defaultOutlineWidth;
}),
mouseCursor: mouseCursor,
materialTapTargetSize: materialTapTargetSize,
focusColor: focusColor,
hoverColor: hoverColor,
splashRadius: splashRadius,
thumbIcon: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return const Icon(Icons.add);
}
return const Icon(Icons.access_alarm);
}),
),
),
);
}
// Switch.
await tester.pumpWidget(buildSwitch());
await tester.pumpAndSettle();
expect(
_getSwitchMaterial(tester),
paints
..rrect(color: defaultTrackColor)
..rrect(color: defaultOutlineColor, strokeWidth: defaultOutlineWidth)
..rrect(color: defaultThumbColor)..paragraph(offset: const Offset(12, 12))
);
// Size from MaterialTapTargetSize.shrinkWrap.
expect(tester.getSize(find.byType(Switch)), const Size(60.0, 40.0));
// Selected switch.
await tester.pumpWidget(buildSwitch(selected: true));
await tester.pumpAndSettle();
expect(
_getSwitchMaterial(tester),
paints
..rrect(color: selectedTrackColor)..rrect(color: selectedOutlineColor, strokeWidth: selectedOutlineWidth)
..rrect(color: selectedThumbColor)
);
// Switch with hover.
await tester.pumpWidget(buildSwitch());
await _pointGestureToSwitch(tester);
await tester.pumpAndSettle();
expect(RendererBinding.instance.mouseTracker.debugDeviceActiveCursor(1), SystemMouseCursors.text);
expect(_getSwitchMaterial(tester), paints..circle(color: hoverColor));
// Switch with focus.
await tester.pumpWidget(buildSwitch(autofocus: true));
await tester.pumpAndSettle();
expect(_getSwitchMaterial(tester), paints..circle(color: focusColor, radius: splashRadius));
});
testWidgets('Material2 - Switch active and inactive properties are taken over the theme values', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
const Color themeDefaultThumbColor = Color(0xfffffff0);
const Color themeSelectedThumbColor = Color(0xfffffff1);
const Color themeDefaultTrackColor = Color(0xfffffff2);
const Color themeSelectedTrackColor = Color(0xfffffff3);
const Color defaultThumbColor = Color(0xffffff0f);
const Color selectedThumbColor = Color(0xffffff1f);
const Color defaultTrackColor = Color(0xffffff2f);
const Color selectedTrackColor = Color(0xffffff3f);
final ThemeData themeData = ThemeData(
useMaterial3: false,
switchTheme: SwitchThemeData(
thumbColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return themeSelectedThumbColor;
}
return themeDefaultThumbColor;
}),
trackColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return themeSelectedTrackColor;
}
return themeDefaultTrackColor;
}),
),
);
Widget buildSwitch({bool selected = false, bool autofocus = false}) {
return MaterialApp(
theme: themeData,
home: Scaffold(
body: Switch(
value: selected,
onChanged: (bool value) {},
autofocus: autofocus,
activeColor: selectedThumbColor,
inactiveThumbColor: defaultThumbColor,
activeTrackColor: selectedTrackColor,
inactiveTrackColor: defaultTrackColor,
),
),
);
}
// Unselected switch.
await tester.pumpWidget(buildSwitch());
await tester.pumpAndSettle();
expect(
_getSwitchMaterial(tester),
paints
..rrect(color: defaultTrackColor)
..rrect()
..rrect()
..rrect()
..rrect()
..rrect(color: defaultThumbColor)
);
// Selected switch.
await tester.pumpWidget(buildSwitch(selected: true));
await tester.pumpAndSettle();
expect(
_getSwitchMaterial(tester),
paints
..rrect(color: selectedTrackColor)
..rrect()
..rrect()
..rrect()
..rrect()
..rrect(color: selectedThumbColor)
);
});
testWidgets('Material3 - Switch active and inactive properties are taken over the theme values', (WidgetTester tester) async {
tester.binding.focusManager.highlightStrategy = FocusHighlightStrategy.alwaysTraditional;
const Color themeDefaultThumbColor = Color(0xfffffff0);
const Color themeSelectedThumbColor = Color(0xfffffff1);
const Color themeDefaultTrackColor = Color(0xfffffff2);
const Color themeSelectedTrackColor = Color(0xfffffff3);
const Color defaultThumbColor = Color(0xffffff0f);
const Color selectedThumbColor = Color(0xffffff1f);
const Color defaultTrackColor = Color(0xffffff2f);
const Color selectedTrackColor = Color(0xffffff3f);
final ThemeData themeData = ThemeData(
useMaterial3: true,
switchTheme: SwitchThemeData(
thumbColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return themeSelectedThumbColor;
}
return themeDefaultThumbColor;
}),
trackColor: MaterialStateProperty.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.selected)) {
return themeSelectedTrackColor;
}
return themeDefaultTrackColor;
}),
),
);
Widget buildSwitch({bool selected = false, bool autofocus = false}) {
return MaterialApp(
theme: themeData,
home: Scaffold(
body: Switch(
value: selected,
onChanged: (bool value) {},
autofocus: autofocus,
activeColor: selectedThumbColor,
inactiveThumbColor: defaultThumbColor,
activeTrackColor: selectedTrackColor,
inactiveTrackColor: defaultTrackColor,
),
),
);
}
// Unselected switch.
await tester.pumpWidget(buildSwitch());
await tester.pumpAndSettle();
expect(
_getSwitchMaterial(tester),
paints
..rrect(color: defaultTrackColor)
..rrect(color: themeData.colorScheme.outline)
..rrect(color: defaultThumbColor)
);
// Selected switch.
await tester.pumpWidget(buildSwitch(selected: true));
await tester.pumpAndSettle();
expect(
_getSwitchMaterial(tester),
paints
..rrect(color: selectedTrackColor)
..rrect()
..rrect(color: selectedThumbColor)
);
});
testWidgets('Material2 - Switch theme overlay color resolves in active/pressed states', (WidgetTester tester) async {
const Color activePressedOverlayColor = Color(0xFF000001);
const Color inactivePressedOverlayColor = Color(0xFF000002);
Color? getOverlayColor(Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
if (states.contains(MaterialState.selected)) {
return activePressedOverlayColor;
}
return inactivePressedOverlayColor;
}
return null;
}
const double splashRadius = 24.0;
final ThemeData themeData = ThemeData(
useMaterial3: false,
switchTheme: SwitchThemeData(
overlayColor: MaterialStateProperty.resolveWith(getOverlayColor),
splashRadius: splashRadius,
),
);
Widget buildSwitch({required bool active}) {
return MaterialApp(
theme: themeData,
home: Scaffold(
body: Switch(
value: active,
onChanged: (_) { },
),
),
);
}
await tester.pumpWidget(buildSwitch(active: false));
await tester.press(find.byType(Switch));
await tester.pumpAndSettle();
expect(
_getSwitchMaterial(tester),
paints
..rrect()
..circle(
color: inactivePressedOverlayColor,
radius: splashRadius,
),
reason: 'Inactive pressed Switch should have overlay color: $inactivePressedOverlayColor',
);
await tester.pumpWidget(buildSwitch(active: true));
await tester.press(find.byType(Switch));
await tester.pumpAndSettle();
expect(
_getSwitchMaterial(tester),
paints
..rrect()
..circle(
color: activePressedOverlayColor,
radius: splashRadius,
),
reason: 'Active pressed Switch should have overlay color: $activePressedOverlayColor',
);
});
testWidgets('Material3 - Switch theme overlay color resolves in active/pressed states', (WidgetTester tester) async {
const Color activePressedOverlayColor = Color(0xFF000001);
const Color inactivePressedOverlayColor = Color(0xFF000002);
Color? getOverlayColor(Set<MaterialState> states) {
if (states.contains(MaterialState.pressed)) {
if (states.contains(MaterialState.selected)) {
return activePressedOverlayColor;
}
return inactivePressedOverlayColor;
}
return null;
}
const double splashRadius = 24.0;
final ThemeData themeData = ThemeData(
useMaterial3: true,
switchTheme: SwitchThemeData(
overlayColor: MaterialStateProperty.resolveWith(getOverlayColor),
splashRadius: splashRadius,
),
);
Widget buildSwitch({required bool active}) {
return MaterialApp(
theme: themeData,
home: Scaffold(
body: Switch(
value: active,
onChanged: (_) { },
),
),
);
}
await tester.pumpWidget(buildSwitch(active: false));
await tester.press(find.byType(Switch));
await tester.pumpAndSettle();
expect(
_getSwitchMaterial(tester),
(paints
..rrect()
..rrect())
..circle(
color: inactivePressedOverlayColor,
radius: splashRadius,
),
reason: 'Inactive pressed Switch should have overlay color: $inactivePressedOverlayColor',
);
await tester.pumpWidget(buildSwitch(active: true));
await tester.press(find.byType(Switch));
await tester.pumpAndSettle();
expect(
_getSwitchMaterial(tester),
paints
..rrect()
..circle(
color: activePressedOverlayColor,
radius: splashRadius,
),
reason: 'Active pressed Switch should have overlay color: $activePressedOverlayColor',
);
});
testWidgets('Material2 - Local SwitchTheme can override global SwitchTheme', (WidgetTester tester) async {
const Color globalThemeThumbColor = Color(0xfffffff1);
const Color globalThemeTrackColor = Color(0xfffffff2);
const Color globalThemeOutlineColor = Color(0xfffffff3);
const double globalThemeOutlineWidth = 6.0;
const Color localThemeThumbColor = Color(0xffff0000);
const Color localThemeTrackColor = Color(0xffff0000);
const Color localThemeOutlineColor = Color(0xffff0000);
const double localThemeOutlineWidth = 4.0;
final ThemeData themeData = ThemeData(
useMaterial3: false,
switchTheme: const SwitchThemeData(
thumbColor: MaterialStatePropertyAll<Color>(globalThemeThumbColor),
trackColor: MaterialStatePropertyAll<Color>(globalThemeTrackColor),
trackOutlineColor: MaterialStatePropertyAll<Color>(globalThemeOutlineColor),
trackOutlineWidth: MaterialStatePropertyAll<double>(globalThemeOutlineWidth),
),
);
Widget buildSwitch({bool selected = false, bool autofocus = false}) {
return MaterialApp(
theme: themeData,
home: Scaffold(
body: SwitchTheme(
data: const SwitchThemeData(
thumbColor: MaterialStatePropertyAll<Color>(localThemeThumbColor),
trackColor: MaterialStatePropertyAll<Color>(localThemeTrackColor),
trackOutlineColor: MaterialStatePropertyAll<Color>(localThemeOutlineColor),
trackOutlineWidth: MaterialStatePropertyAll<double>(localThemeOutlineWidth)
),
child: Switch(
value: selected,
onChanged: (bool value) {},
autofocus: autofocus,
),
),
),
);
}
await tester.pumpWidget(buildSwitch(selected: true));
await tester.pumpAndSettle();
expect(
_getSwitchMaterial(tester),
paints
..rrect(color: localThemeTrackColor)
..rrect(color: localThemeOutlineColor, strokeWidth: localThemeOutlineWidth)
..rrect()
..rrect()
..rrect()
..rrect(color: localThemeThumbColor)
);
});
testWidgets('Material3 - Local SwitchTheme can override global SwitchTheme', (WidgetTester tester) async {
const Color globalThemeThumbColor = Color(0xfffffff1);
const Color globalThemeTrackColor = Color(0xfffffff2);
const Color globalThemeOutlineColor = Color(0xfffffff3);
const double globalThemeOutlineWidth = 6.0;
const Color localThemeThumbColor = Color(0xffff0000);
const Color localThemeTrackColor = Color(0xffff0000);
const Color localThemeOutlineColor = Color(0xffff0000);
const double localThemeOutlineWidth = 4.0;
final ThemeData themeData = ThemeData(
useMaterial3: true,
switchTheme: const SwitchThemeData(
thumbColor: MaterialStatePropertyAll<Color>(globalThemeThumbColor),
trackColor: MaterialStatePropertyAll<Color>(globalThemeTrackColor),
trackOutlineColor: MaterialStatePropertyAll<Color>(globalThemeOutlineColor),
trackOutlineWidth: MaterialStatePropertyAll<double>(globalThemeOutlineWidth),
),
);
Widget buildSwitch({bool selected = false, bool autofocus = false}) {
return MaterialApp(
theme: themeData,
home: Scaffold(
body: SwitchTheme(
data: const SwitchThemeData(
thumbColor: MaterialStatePropertyAll<Color>(localThemeThumbColor),
trackColor: MaterialStatePropertyAll<Color>(localThemeTrackColor),
trackOutlineColor: MaterialStatePropertyAll<Color>(localThemeOutlineColor),
trackOutlineWidth: MaterialStatePropertyAll<double>(localThemeOutlineWidth)
),
child: Switch(
value: selected,
onChanged: (bool value) {},
autofocus: autofocus,
),
),
),
);
}
await tester.pumpWidget(buildSwitch(selected: true));
await tester.pumpAndSettle();
expect(
_getSwitchMaterial(tester),
paints
..rrect(color: localThemeTrackColor)
..rrect(color: localThemeOutlineColor, strokeWidth: localThemeOutlineWidth)
..rrect(color: localThemeThumbColor)
);
});
}
Future<void> _pointGestureToSwitch(WidgetTester tester) async {
final TestGesture gesture = await tester.createGesture(kind: PointerDeviceKind.mouse);
await gesture.addPointer();
addTearDown(gesture.removePointer);
await gesture.moveTo(tester.getCenter(find.byType(Switch)));
}
MaterialInkController? _getSwitchMaterial(WidgetTester tester) {
return Material.of(tester.element(find.byType(Switch)));
}
| flutter/packages/flutter/test/material/switch_theme_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/switch_theme_test.dart",
"repo_id": "flutter",
"token_count": 16059
} | 695 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('TextSelectionThemeData copyWith, ==, hashCode basics', () {
expect(const TextSelectionThemeData(), const TextSelectionThemeData().copyWith());
expect(const TextSelectionThemeData().hashCode, const TextSelectionThemeData().copyWith().hashCode);
});
test('TextSelectionThemeData lerp special cases', () {
expect(TextSelectionThemeData.lerp(null, null, 0), null);
const TextSelectionThemeData data = TextSelectionThemeData();
expect(identical(TextSelectionThemeData.lerp(data, data, 0.5), data), true);
});
test('TextSelectionThemeData null fields by default', () {
const TextSelectionThemeData theme = TextSelectionThemeData();
expect(theme.cursorColor, null);
expect(theme.selectionColor, null);
expect(theme.selectionHandleColor, null);
});
testWidgets('Default TextSelectionThemeData debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const TextSelectionThemeData().debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description, <String>[]);
});
testWidgets('TextSelectionThemeData implements debugFillProperties', (WidgetTester tester) async {
final DiagnosticPropertiesBuilder builder = DiagnosticPropertiesBuilder();
const TextSelectionThemeData(
cursorColor: Color(0xffeeffaa),
selectionColor: Color(0x88888888),
selectionHandleColor: Color(0xaabbccdd),
).debugFillProperties(builder);
final List<String> description = builder.properties
.where((DiagnosticsNode node) => !node.isFiltered(DiagnosticLevel.info))
.map((DiagnosticsNode node) => node.toString())
.toList();
expect(description, <String>[
'cursorColor: Color(0xffeeffaa)',
'selectionColor: Color(0x88888888)',
'selectionHandleColor: Color(0xaabbccdd)',
]);
});
testWidgets('Material2 - Empty textSelectionTheme will use defaults', (WidgetTester tester) async {
final ThemeData theme = ThemeData(useMaterial3: false);
const Color defaultCursorColor = Color(0xff2196f3);
const Color defaultSelectionColor = Color(0x662196f3);
const Color defaultSelectionHandleColor = Color(0xff2196f3);
EditableText.debugDeterministicCursor = true;
addTearDown(() {
EditableText.debugDeterministicCursor = false;
});
// Test TextField's cursor & selection color.
await tester.pumpWidget(
MaterialApp(
theme: theme,
home: const Material(
child: TextField(autofocus: true),
),
),
);
await tester.pump();
await tester.pumpAndSettle();
final EditableTextState editableTextState = tester.firstState(find.byType(EditableText));
final RenderEditable renderEditable = editableTextState.renderEditable;
expect(renderEditable.cursorColor, defaultCursorColor);
expect(renderEditable.selectionColor, defaultSelectionColor);
// Test the selection handle color.
await tester.pumpWidget(
MaterialApp(
theme: theme,
home: Material(
child: Builder(
builder: (BuildContext context) {
return materialTextSelectionControls.buildHandle(
context,
TextSelectionHandleType.left,
10.0,
);
},
),
),
),
);
await tester.pumpAndSettle();
final RenderBox handle = tester.firstRenderObject<RenderBox>(find.byType(CustomPaint));
expect(handle, paints..path(color: defaultSelectionHandleColor));
});
testWidgets('Material3 - Empty textSelectionTheme will use defaults', (WidgetTester tester) async {
final ThemeData theme = ThemeData(useMaterial3: true);
final Color defaultCursorColor = theme.colorScheme.primary;
final Color defaultSelectionColor = theme.colorScheme.primary.withOpacity(0.40);
final Color defaultSelectionHandleColor = theme.colorScheme.primary;
EditableText.debugDeterministicCursor = true;
addTearDown(() {
EditableText.debugDeterministicCursor = false;
});
// Test TextField's cursor & selection color.
await tester.pumpWidget(
MaterialApp(
theme: theme,
home: const Material(
child: TextField(autofocus: true),
),
),
);
await tester.pump();
await tester.pumpAndSettle();
final EditableTextState editableTextState = tester.firstState(find.byType(EditableText));
final RenderEditable renderEditable = editableTextState.renderEditable;
expect(renderEditable.cursorColor, defaultCursorColor);
expect(renderEditable.selectionColor, defaultSelectionColor);
// Test the selection handle color.
await tester.pumpWidget(
MaterialApp(
theme: theme,
home: Material(
child: Builder(
builder: (BuildContext context) {
return materialTextSelectionControls.buildHandle(
context,
TextSelectionHandleType.left,
10.0,
);
},
),
),
),
);
await tester.pumpAndSettle();
final RenderBox handle = tester.firstRenderObject<RenderBox>(find.byType(CustomPaint));
expect(handle, paints..path(color: defaultSelectionHandleColor));
});
testWidgets('ThemeData.textSelectionTheme will be used if provided', (WidgetTester tester) async {
const TextSelectionThemeData textSelectionTheme = TextSelectionThemeData(
cursorColor: Color(0xffaabbcc),
selectionColor: Color(0x88888888),
selectionHandleColor: Color(0x00ccbbaa),
);
final ThemeData theme = ThemeData.fallback().copyWith(
textSelectionTheme: textSelectionTheme,
);
EditableText.debugDeterministicCursor = true;
addTearDown(() {
EditableText.debugDeterministicCursor = false;
});
// Test TextField's cursor & selection color.
await tester.pumpWidget(
MaterialApp(
theme: theme,
home: const Material(
child: TextField(autofocus: true),
),
),
);
await tester.pump();
final EditableTextState editableTextState = tester.firstState(find.byType(EditableText));
final RenderEditable renderEditable = editableTextState.renderEditable;
expect(renderEditable.cursorColor, textSelectionTheme.cursorColor);
expect(renderEditable.selectionColor, textSelectionTheme.selectionColor);
// Test the selection handle color.
await tester.pumpWidget(
MaterialApp(
theme: theme,
home: Material(
child: Builder(
builder: (BuildContext context) {
return materialTextSelectionControls.buildHandle(
context,
TextSelectionHandleType.left,
10.0,
);
},
),
),
),
);
await tester.pumpAndSettle();
final RenderBox handle = tester.firstRenderObject<RenderBox>(find.byType(CustomPaint));
expect(handle, paints..path(color: textSelectionTheme.selectionHandleColor));
});
testWidgets('TextSelectionTheme widget will override ThemeData.textSelectionTheme', (WidgetTester tester) async {
const TextSelectionThemeData defaultTextSelectionTheme = TextSelectionThemeData(
cursorColor: Color(0xffaabbcc),
selectionColor: Color(0x88888888),
selectionHandleColor: Color(0x00ccbbaa),
);
final ThemeData theme = ThemeData.fallback().copyWith(
textSelectionTheme: defaultTextSelectionTheme,
);
const TextSelectionThemeData widgetTextSelectionTheme = TextSelectionThemeData(
cursorColor: Color(0xffddeeff),
selectionColor: Color(0x44444444),
selectionHandleColor: Color(0x00ffeedd),
);
EditableText.debugDeterministicCursor = true;
addTearDown(() {
EditableText.debugDeterministicCursor = false;
});
// Test TextField's cursor & selection color.
await tester.pumpWidget(
MaterialApp(
theme: theme,
home: const Material(
child: TextSelectionTheme(
data: widgetTextSelectionTheme,
child: TextField(autofocus: true),
),
),
),
);
await tester.pump();
final EditableTextState editableTextState = tester.firstState(find.byType(EditableText));
final RenderEditable renderEditable = editableTextState.renderEditable;
expect(renderEditable.cursorColor, widgetTextSelectionTheme.cursorColor);
expect(renderEditable.selectionColor, widgetTextSelectionTheme.selectionColor);
// Test the selection handle color.
await tester.pumpWidget(
MaterialApp(
theme: theme,
home: Material(
child: TextSelectionTheme(
data: widgetTextSelectionTheme,
child: Builder(
builder: (BuildContext context) {
return materialTextSelectionControls.buildHandle(
context,
TextSelectionHandleType.left,
10.0,
);
},
),
),
),
),
);
await tester.pumpAndSettle();
final RenderBox handle = tester.firstRenderObject<RenderBox>(find.byType(CustomPaint));
expect(handle, paints..path(color: widgetTextSelectionTheme.selectionHandleColor));
});
testWidgets('TextField parameters will override theme settings', (WidgetTester tester) async {
const TextSelectionThemeData defaultTextSelectionTheme = TextSelectionThemeData(
cursorColor: Color(0xffaabbcc),
selectionHandleColor: Color(0x00ccbbaa),
);
final ThemeData theme = ThemeData.fallback().copyWith(
textSelectionTheme: defaultTextSelectionTheme,
);
const TextSelectionThemeData widgetTextSelectionTheme = TextSelectionThemeData(
cursorColor: Color(0xffddeeff),
selectionHandleColor: Color(0x00ffeedd),
);
const Color cursorColor = Color(0x88888888);
// Test TextField's cursor color.
await tester.pumpWidget(
MaterialApp(
theme: theme,
home: const Material(
child: TextSelectionTheme(
data: widgetTextSelectionTheme,
child: TextField(cursorColor: cursorColor),
),
),
),
);
await tester.pumpAndSettle();
final EditableTextState editableTextState = tester.firstState(find.byType(EditableText));
final RenderEditable renderEditable = editableTextState.renderEditable;
expect(renderEditable.cursorColor, cursorColor.withAlpha(0));
// Test SelectableText's cursor color.
await tester.pumpWidget(
MaterialApp(
theme: theme,
home: const Material(
child: TextSelectionTheme(
data: widgetTextSelectionTheme,
child: SelectableText('foobar', cursorColor: cursorColor),
),
),
),
);
await tester.pumpAndSettle();
final EditableTextState selectableTextState = tester.firstState(find.byType(EditableText));
final RenderEditable renderSelectable = selectableTextState.renderEditable;
expect(renderSelectable.cursorColor, cursorColor.withAlpha(0));
});
testWidgets('TextSelectionThem overrides DefaultSelectionStyle', (WidgetTester tester) async {
const Color themeSelectionColor = Color(0xffaabbcc);
const Color themeCursorColor = Color(0x00ccbbaa);
const Color defaultSelectionColor = Color(0xffaa1111);
const Color defaultCursorColor = Color(0x00cc2222);
final Key defaultSelectionStyle = UniqueKey();
final Key themeStyle = UniqueKey();
// Test TextField's cursor color.
await tester.pumpWidget(
MaterialApp(
home: DefaultSelectionStyle(
selectionColor: defaultSelectionColor,
cursorColor: defaultCursorColor,
child: Container(
key: defaultSelectionStyle,
child: TextSelectionTheme(
data: const TextSelectionThemeData(
selectionColor: themeSelectionColor,
cursorColor: themeCursorColor,
),
child: Placeholder(
key: themeStyle,
),
),
)
),
),
);
final BuildContext defaultSelectionStyleContext = tester.element(find.byKey(defaultSelectionStyle));
DefaultSelectionStyle style = DefaultSelectionStyle.of(defaultSelectionStyleContext);
expect(style.selectionColor, defaultSelectionColor);
expect(style.cursorColor, defaultCursorColor);
final BuildContext themeStyleContext = tester.element(find.byKey(themeStyle));
style = DefaultSelectionStyle.of(themeStyleContext);
expect(style.selectionColor, themeSelectionColor);
expect(style.cursorColor, themeCursorColor);
});
}
| flutter/packages/flutter/test/material/text_selection_theme_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/text_selection_theme_test.dart",
"repo_id": "flutter",
"token_count": 5071
} | 696 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/semantics_tester.dart';
const Key avatarA = Key('A');
const Key avatarC = Key('C');
const Key avatarD = Key('D');
Future<void> pumpTestWidget(
WidgetTester tester, {
bool withName = true,
bool withEmail = true,
bool withOnDetailsPressedHandler = true,
Size otherAccountsPictureSize = const Size.square(40.0),
Size currentAccountPictureSize = const Size.square(72.0),
Color? primaryColor,
Color? colorSchemePrimary,
}) async {
await tester.pumpWidget(
MaterialApp(
theme: ThemeData(
primaryColor: primaryColor,
colorScheme: const ColorScheme.light().copyWith(primary: colorSchemePrimary),
),
home: MediaQuery(
data: const MediaQueryData(
padding: EdgeInsets.only(
left: 10.0,
top: 20.0,
right: 30.0,
bottom: 40.0,
),
),
child: Material(
child: Center(
child: UserAccountsDrawerHeader(
onDetailsPressed: withOnDetailsPressedHandler ? () { } : null,
currentAccountPictureSize: currentAccountPictureSize,
otherAccountsPicturesSize: otherAccountsPictureSize,
currentAccountPicture: const ExcludeSemantics(
child: CircleAvatar(
key: avatarA,
child: Text('A'),
),
),
otherAccountsPictures: const <Widget>[
CircleAvatar(
child: Text('B'),
),
CircleAvatar(
key: avatarC,
child: Text('C'),
),
CircleAvatar(
key: avatarD,
child: Text('D'),
),
CircleAvatar(
child: Text('E'),
),
],
accountName: withName ? const Text('name') : null,
accountEmail: withEmail ? const Text('email') : null,
),
),
),
),
),
);
}
void main() {
// Find the exact transform which is the descendant of [UserAccountsDrawerHeader].
final Finder findTransform = find.descendant(
of: find.byType(UserAccountsDrawerHeader),
matching: find.byType(Transform),
);
testWidgets('UserAccountsDrawerHeader inherits ColorScheme.primary', (WidgetTester tester) async {
const Color primaryColor = Color(0xff00ff00);
const Color colorSchemePrimary = Color(0xff0000ff);
await pumpTestWidget(tester, primaryColor: primaryColor, colorSchemePrimary: colorSchemePrimary);
final BoxDecoration? boxDecoration = tester.widget<DrawerHeader>(
find.byType(DrawerHeader),
).decoration as BoxDecoration?;
expect(boxDecoration?.color == primaryColor, false);
expect(boxDecoration?.color == colorSchemePrimary, true);
});
testWidgets('UserAccountsDrawerHeader test', (WidgetTester tester) async {
await pumpTestWidget(tester);
expect(find.text('A'), findsOneWidget);
expect(find.text('B'), findsOneWidget);
expect(find.text('C'), findsOneWidget);
expect(find.text('D'), findsOneWidget);
expect(find.text('E'), findsNothing);
expect(find.text('name'), findsOneWidget);
expect(find.text('email'), findsOneWidget);
RenderBox box = tester.renderObject(find.byKey(avatarA));
expect(box.size.width, equals(72.0));
expect(box.size.height, equals(72.0));
box = tester.renderObject(find.byKey(avatarC));
expect(box.size.width, equals(40.0));
expect(box.size.height, equals(40.0));
// Verify height = height + top padding + bottom margin + bottom edge)
box = tester.renderObject(find.byType(UserAccountsDrawerHeader));
expect(box.size.height, equals(160.0 + 20.0 + 8.0 + 1.0));
final Offset topLeft = tester.getTopLeft(find.byType(UserAccountsDrawerHeader));
final Offset topRight = tester.getTopRight(find.byType(UserAccountsDrawerHeader));
final Offset avatarATopLeft = tester.getTopLeft(find.byKey(avatarA));
final Offset avatarDTopRight = tester.getTopRight(find.byKey(avatarD));
final Offset avatarCTopRight = tester.getTopRight(find.byKey(avatarC));
expect(avatarATopLeft.dx - topLeft.dx, equals(16.0 + 10.0)); // left padding
expect(avatarATopLeft.dy - topLeft.dy, equals(16.0 + 20.0)); // add top padding
expect(topRight.dx - avatarDTopRight.dx, equals(16.0 + 30.0)); // right padding
expect(avatarDTopRight.dy - topRight.dy, equals(16.0 + 20.0)); // add top padding
expect(avatarDTopRight.dx - avatarCTopRight.dx, equals(40.0 + 16.0)); // size + space between
});
testWidgets('UserAccountsDrawerHeader change default size test', (WidgetTester tester) async {
const Size currentAccountPictureSize = Size.square(60.0);
const Size otherAccountsPictureSize = Size.square(30.0);
await pumpTestWidget(
tester,
currentAccountPictureSize: currentAccountPictureSize,
otherAccountsPictureSize: otherAccountsPictureSize,
);
final RenderBox currentAccountRenderBox = tester.renderObject(find.byKey(avatarA));
final RenderBox otherAccountRenderBox = tester.renderObject(find.byKey(avatarC));
expect(currentAccountRenderBox.size, currentAccountPictureSize);
expect(otherAccountRenderBox.size, otherAccountsPictureSize);
});
testWidgets('UserAccountsDrawerHeader icon rotation test', (WidgetTester tester) async {
await pumpTestWidget(tester);
Transform transformWidget = tester.firstWidget(findTransform);
// Icon is right side up.
expect(transformWidget.transform.getRotation()[0], 1.0);
expect(transformWidget.transform.getRotation()[4], 1.0);
await tester.tap(find.byType(Icon));
await tester.pump();
await tester.pump(const Duration(milliseconds: 10));
expect(tester.hasRunningAnimations, isTrue);
await tester.pumpAndSettle();
await tester.pump();
transformWidget = tester.firstWidget(findTransform);
// Icon has rotated 180 degrees.
expect(transformWidget.transform.getRotation()[0], -1.0);
expect(transformWidget.transform.getRotation()[4], -1.0);
await tester.tap(find.byType(Icon));
await tester.pump();
await tester.pump(const Duration(milliseconds: 10));
expect(tester.hasRunningAnimations, isTrue);
await tester.pumpAndSettle();
await tester.pump();
transformWidget = tester.firstWidget(findTransform);
// Icon has rotated 180 degrees back to the original position.
expect(transformWidget.transform.getRotation()[0], 1.0);
expect(transformWidget.transform.getRotation()[4], 1.0);
});
// Regression test for https://github.com/flutter/flutter/issues/25801.
testWidgets('UserAccountsDrawerHeader icon does not rotate after setState', (WidgetTester tester) async {
late StateSetter testSetState;
await tester.pumpWidget(MaterialApp(
home: Material(
child: StatefulBuilder(
builder: (BuildContext context, StateSetter setState) {
testSetState = setState;
return UserAccountsDrawerHeader(
onDetailsPressed: () { },
accountName: const Text('name'),
accountEmail: const Text('email'),
);
},
),
),
));
Transform transformWidget = tester.firstWidget(findTransform);
// Icon is right side up.
expect(transformWidget.transform.getRotation()[0], 1.0);
expect(transformWidget.transform.getRotation()[4], 1.0);
testSetState(() { });
await tester.pump(const Duration(milliseconds: 10));
expect(tester.hasRunningAnimations, isFalse);
expect(await tester.pumpAndSettle(), 1);
transformWidget = tester.firstWidget(findTransform);
// Icon has not rotated.
expect(transformWidget.transform.getRotation()[0], 1.0);
expect(transformWidget.transform.getRotation()[4], 1.0);
});
testWidgets('UserAccountsDrawerHeader icon rotation test speeeeeedy', (WidgetTester tester) async {
await pumpTestWidget(tester);
Transform transformWidget = tester.firstWidget(findTransform);
// Icon is right side up.
expect(transformWidget.transform.getRotation()[0], 1.0);
expect(transformWidget.transform.getRotation()[4], 1.0);
// Icon starts to rotate down.
await tester.tap(find.byType(Icon));
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
expect(tester.hasRunningAnimations, isTrue);
// Icon starts to rotate up mid animation.
await tester.tap(find.byType(Icon));
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
expect(tester.hasRunningAnimations, isTrue);
// Icon starts to rotate down again still mid animation.
await tester.tap(find.byType(Icon));
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
expect(tester.hasRunningAnimations, isTrue);
// Icon starts to rotate up to its original position mid animation.
await tester.tap(find.byType(Icon));
await tester.pump();
await tester.pump(const Duration(milliseconds: 100));
expect(tester.hasRunningAnimations, isTrue);
await tester.pumpAndSettle();
await tester.pump();
transformWidget = tester.firstWidget(findTransform);
// Icon has rotated 180 degrees back to the original position.
expect(transformWidget.transform.getRotation()[0], 1.0);
expect(transformWidget.transform.getRotation()[4], 1.0);
});
testWidgets('UserAccountsDrawerHeader icon color changes', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
home: Material(
child: UserAccountsDrawerHeader(
onDetailsPressed: () {},
accountName: const Text('name'),
accountEmail: const Text('email'),
),
),
));
Icon iconWidget = tester.firstWidget(find.byType(Icon));
// Default icon color is white.
expect(iconWidget.color, Colors.white);
const Color arrowColor = Colors.red;
await tester.pumpWidget(MaterialApp(
home: Material(
child: UserAccountsDrawerHeader(
onDetailsPressed: () { },
accountName: const Text('name'),
accountEmail: const Text('email'),
arrowColor: arrowColor,
),
),
));
iconWidget = tester.firstWidget(find.byType(Icon));
expect(iconWidget.color, arrowColor);
});
testWidgets('UserAccountsDrawerHeader null parameters LTR', (WidgetTester tester) async {
Widget buildFrame({
Widget? currentAccountPicture,
List<Widget>? otherAccountsPictures,
Widget? accountName,
Widget? accountEmail,
VoidCallback? onDetailsPressed,
EdgeInsets? margin,
}) {
return MaterialApp(
home: Material(
child: Center(
child: UserAccountsDrawerHeader(
currentAccountPicture: currentAccountPicture,
otherAccountsPictures: otherAccountsPictures,
accountName: accountName,
accountEmail: accountEmail,
onDetailsPressed: onDetailsPressed,
margin: margin,
),
),
),
);
}
await tester.pumpWidget(buildFrame());
final RenderBox box = tester.renderObject(find.byType(UserAccountsDrawerHeader));
expect(box.size.height, equals(160.0 + 1.0)); // height + bottom edge)
expect(find.byType(Icon), findsNothing);
await tester.pumpWidget(buildFrame(
onDetailsPressed: () { },
));
expect(find.byType(Icon), findsOneWidget);
await tester.pumpWidget(buildFrame(
accountName: const Text('accountName'),
onDetailsPressed: () { },
));
expect(
tester.getCenter(find.text('accountName')).dy,
tester.getCenter(find.byType(Icon)).dy,
);
expect(
tester.getCenter(find.text('accountName')).dx,
lessThan(tester.getCenter(find.byType(Icon)).dx),
);
await tester.pumpWidget(buildFrame(
accountEmail: const Text('accountEmail'),
onDetailsPressed: () { },
));
expect(
tester.getCenter(find.text('accountEmail')).dy,
tester.getCenter(find.byType(Icon)).dy,
);
expect(
tester.getCenter(find.text('accountEmail')).dx,
lessThan(tester.getCenter(find.byType(Icon)).dx),
);
await tester.pumpWidget(buildFrame(
accountName: const Text('accountName'),
accountEmail: const Text('accountEmail'),
onDetailsPressed: () { },
));
expect(
tester.getCenter(find.text('accountEmail')).dy,
tester.getCenter(find.byType(Icon)).dy,
);
expect(
tester.getCenter(find.text('accountEmail')).dx,
lessThan(tester.getCenter(find.byType(Icon)).dx),
);
expect(
tester.getBottomLeft(find.text('accountEmail')).dy,
greaterThan(tester.getBottomLeft(find.text('accountName')).dy),
);
expect(
tester.getBottomLeft(find.text('accountEmail')).dx,
tester.getBottomLeft(find.text('accountName')).dx,
);
await tester.pumpWidget(buildFrame(
currentAccountPicture: const CircleAvatar(child: Text('A')),
));
expect(find.text('A'), findsOneWidget);
await tester.pumpWidget(buildFrame(
otherAccountsPictures: <Widget>[const CircleAvatar(child: Text('A'))],
));
expect(find.text('A'), findsOneWidget);
const Key avatarA = Key('A');
await tester.pumpWidget(buildFrame(
currentAccountPicture: const CircleAvatar(key: avatarA, child: Text('A')),
accountName: const Text('accountName'),
));
expect(
tester.getBottomLeft(find.byKey(avatarA)).dx,
tester.getBottomLeft(find.text('accountName')).dx,
);
expect(
tester.getBottomLeft(find.text('accountName')).dy,
greaterThan(tester.getBottomLeft(find.byKey(avatarA)).dy),
);
});
testWidgets('UserAccountsDrawerHeader null parameters RTL', (WidgetTester tester) async {
Widget buildFrame({
Widget? currentAccountPicture,
List<Widget>? otherAccountsPictures,
Widget? accountName,
Widget? accountEmail,
VoidCallback? onDetailsPressed,
EdgeInsets? margin,
}) {
return MaterialApp(
home: Directionality(
textDirection: TextDirection.rtl,
child: Material(
child: Center(
child: UserAccountsDrawerHeader(
currentAccountPicture: currentAccountPicture,
otherAccountsPictures: otherAccountsPictures,
accountName: accountName,
accountEmail: accountEmail,
onDetailsPressed: onDetailsPressed,
margin: margin,
),
),
),
),
);
}
await tester.pumpWidget(buildFrame());
final RenderBox box = tester.renderObject(find.byType(UserAccountsDrawerHeader));
expect(box.size.height, equals(160.0 + 1.0)); // height + bottom edge)
expect(find.byType(Icon), findsNothing);
await tester.pumpWidget(buildFrame(
onDetailsPressed: () { },
));
expect(find.byType(Icon), findsOneWidget);
await tester.pumpWidget(buildFrame(
accountName: const Text('accountName'),
onDetailsPressed: () { },
));
expect(
tester.getCenter(find.text('accountName')).dy,
tester.getCenter(find.byType(Icon)).dy,
);
expect(
tester.getCenter(find.text('accountName')).dx,
greaterThan(tester.getCenter(find.byType(Icon)).dx),
);
await tester.pumpWidget(buildFrame(
accountEmail: const Text('accountEmail'),
onDetailsPressed: () { },
));
expect(
tester.getCenter(find.text('accountEmail')).dy,
tester.getCenter(find.byType(Icon)).dy,
);
expect(
tester.getCenter(find.text('accountEmail')).dx,
greaterThan(tester.getCenter(find.byType(Icon)).dx),
);
await tester.pumpWidget(buildFrame(
accountName: const Text('accountName'),
accountEmail: const Text('accountEmail'),
onDetailsPressed: () { },
));
expect(
tester.getCenter(find.text('accountEmail')).dy,
tester.getCenter(find.byType(Icon)).dy,
);
expect(
tester.getCenter(find.text('accountEmail')).dx,
greaterThan(tester.getCenter(find.byType(Icon)).dx),
);
expect(
tester.getBottomLeft(find.text('accountEmail')).dy,
greaterThan(tester.getBottomLeft(find.text('accountName')).dy),
);
expect(
tester.getBottomRight(find.text('accountEmail')).dx,
tester.getBottomRight(find.text('accountName')).dx,
);
await tester.pumpWidget(buildFrame(
currentAccountPicture: const CircleAvatar(child: Text('A')),
));
expect(find.text('A'), findsOneWidget);
await tester.pumpWidget(buildFrame(
otherAccountsPictures: <Widget>[const CircleAvatar(child: Text('A'))],
));
expect(find.text('A'), findsOneWidget);
const Key avatarA = Key('A');
await tester.pumpWidget(buildFrame(
currentAccountPicture: const CircleAvatar(key: avatarA, child: Text('A')),
accountName: const Text('accountName'),
));
expect(
tester.getBottomRight(find.byKey(avatarA)).dx,
tester.getBottomRight(find.text('accountName')).dx,
);
expect(
tester.getBottomLeft(find.text('accountName')).dy,
greaterThan(tester.getBottomLeft(find.byKey(avatarA)).dy),
);
});
testWidgets('UserAccountsDrawerHeader provides semantics', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await pumpTestWidget(tester);
expect(
semantics,
hasSemantics(
TestSemantics(
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.scopesRoute],
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isFocusable],
label: 'Signed in\nname\nemail',
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
label: r'B',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: r'C',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: r'D',
textDirection: TextDirection.ltr,
),
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.isButton],
actions: <SemanticsAction>[SemanticsAction.tap],
label: r'Show accounts',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
),
],
),
ignoreId: true, ignoreTransform: true, ignoreRect: true,
),
);
semantics.dispose();
});
testWidgets('alternative account selectors have sufficient tap targets', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
await pumpTestWidget(tester);
expect(tester.getSemantics(find.text('B')), matchesSemantics(
label: 'B',
size: const Size(48.0, 48.0),
));
expect(tester.getSemantics(find.text('C')), matchesSemantics(
label: 'C',
size: const Size(48.0, 48.0),
));
expect(tester.getSemantics(find.text('D')), matchesSemantics(
label: 'D',
size: const Size(48.0, 48.0),
));
handle.dispose();
});
testWidgets('UserAccountsDrawerHeader provides semantics with missing properties', (WidgetTester tester) async {
final SemanticsTester semantics = SemanticsTester(tester);
await pumpTestWidget(
tester,
withEmail: false,
withName: false,
withOnDetailsPressedHandler: false,
);
expect(
semantics,
hasSemantics(
TestSemantics(
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
children: <TestSemantics>[
TestSemantics(
flags: <SemanticsFlag>[SemanticsFlag.scopesRoute],
children: <TestSemantics>[
TestSemantics(
label: 'Signed in',
textDirection: TextDirection.ltr,
children: <TestSemantics>[
TestSemantics(
label: r'B',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: r'C',
textDirection: TextDirection.ltr,
),
TestSemantics(
label: r'D',
textDirection: TextDirection.ltr,
),
],
),
],
),
],
),
],
),
],
),
ignoreId: true, ignoreTransform: true, ignoreRect: true,
),
);
semantics.dispose();
});
}
| flutter/packages/flutter/test/material/user_accounts_drawer_header_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/material/user_accounts_drawer_header_test.dart",
"repo_id": "flutter",
"token_count": 9729
} | 697 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/painting.dart';
import 'package:flutter_test/flutter_test.dart';
import 'common_matchers.dart';
void main() {
test('CircleBorder defaults', () {
const CircleBorder border = CircleBorder();
expect(border.side, BorderSide.none);
expect(border.eccentricity, 0.0);
});
test('CircleBorder getInnerPath and getOuterPath', () {
const Rect circleRect = Rect.fromLTWH(50, 0, 100, 100);
const Rect rect = Rect.fromLTWH(0, 0, 200, 100);
expect(const CircleBorder().getInnerPath(rect).getBounds(), circleRect);
expect(const CircleBorder().getOuterPath(rect).getBounds(), circleRect);
const CircleBorder oval = CircleBorder(eccentricity: 1.0);
expect(oval.getOuterPath(rect).getBounds(), rect);
expect(oval.getInnerPath(rect).getBounds(), rect);
const CircleBorder o10 = CircleBorder(side: BorderSide(width: 10.0), eccentricity: 1.0);
expect(o10.getOuterPath(rect).getBounds(), Offset.zero & const Size(200, 100));
expect(o10.getInnerPath(rect).getBounds(), const Offset(10, 10) & const Size(180, 80));
});
test('CircleBorder copyWith, ==, hashCode', () {
expect(const CircleBorder(), const CircleBorder().copyWith());
expect(const CircleBorder().hashCode, const CircleBorder().copyWith().hashCode);
expect(const CircleBorder(eccentricity: 0.5).hashCode, const CircleBorder().copyWith(eccentricity: 0.5).hashCode);
const BorderSide side = BorderSide(width: 10.0, color: Color(0xff123456));
expect(const CircleBorder().copyWith(side: side), const CircleBorder(side: side));
});
test('CircleBorder', () {
const CircleBorder c10 = CircleBorder(side: BorderSide(width: 10.0));
const CircleBorder c15 = CircleBorder(side: BorderSide(width: 15.0));
const CircleBorder c20 = CircleBorder(side: BorderSide(width: 20.0));
expect(c10.dimensions, const EdgeInsets.all(10.0));
expect(c10.scale(2.0), c20);
expect(c20.scale(0.5), c10);
expect(ShapeBorder.lerp(c10, c20, 0.0), c10);
expect(ShapeBorder.lerp(c10, c20, 0.5), c15);
expect(ShapeBorder.lerp(c10, c20, 1.0), c20);
expect(c10.getInnerPath(Rect.fromCircle(center: Offset.zero, radius: 1.0).inflate(10.0)), isUnitCircle);
expect(c10.getOuterPath(Rect.fromCircle(center: Offset.zero, radius: 1.0)), isUnitCircle);
expect(
(Canvas canvas) => c10.paint(canvas, const Rect.fromLTWH(10.0, 20.0, 30.0, 40.0)),
paints
..circle(x: 25.0, y: 40.0, radius: 10.0, strokeWidth: 10.0),
);
});
}
| flutter/packages/flutter/test/painting/circle_border_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/painting/circle_border_test.dart",
"repo_id": "flutter",
"token_count": 983
} | 698 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui;
import 'package:flutter/foundation.dart';
import 'package:flutter/painting.dart';
import 'package:flutter/scheduler.dart';
import 'package:flutter_test/flutter_test.dart';
import '../rendering/rendering_tester.dart';
import 'mocks_for_image_cache.dart';
void main() {
TestRenderingFlutterBinding.ensureInitialized();
tearDown(() {
imageCache
..clear()
..clearLiveImages()
..maximumSize = 1000
..maximumSizeBytes = 10485760;
});
test('maintains cache size', () async {
imageCache.maximumSize = 3;
final TestImageInfo a = await extractOneFrame(TestImageProvider(1, 1, image: await createTestImage()).resolve(ImageConfiguration.empty)) as TestImageInfo;
expect(a.value, equals(1));
final TestImageInfo b = await extractOneFrame(TestImageProvider(1, 2, image: await createTestImage()).resolve(ImageConfiguration.empty)) as TestImageInfo;
expect(b.value, equals(1));
final TestImageInfo c = await extractOneFrame(TestImageProvider(1, 3, image: await createTestImage()).resolve(ImageConfiguration.empty)) as TestImageInfo;
expect(c.value, equals(1));
final TestImageInfo d = await extractOneFrame(TestImageProvider(1, 4, image: await createTestImage()).resolve(ImageConfiguration.empty)) as TestImageInfo;
expect(d.value, equals(1));
final TestImageInfo e = await extractOneFrame(TestImageProvider(1, 5, image: await createTestImage()).resolve(ImageConfiguration.empty)) as TestImageInfo;
expect(e.value, equals(1));
final TestImageInfo f = await extractOneFrame(TestImageProvider(1, 6, image: await createTestImage()).resolve(ImageConfiguration.empty)) as TestImageInfo;
expect(f.value, equals(1));
expect(f, equals(a));
// cache still only has one entry in it: 1(1)
final TestImageInfo g = await extractOneFrame(TestImageProvider(2, 7, image: await createTestImage()).resolve(ImageConfiguration.empty)) as TestImageInfo;
expect(g.value, equals(7));
// cache has two entries in it: 1(1), 2(7)
final TestImageInfo h = await extractOneFrame(TestImageProvider(1, 8, image: await createTestImage()).resolve(ImageConfiguration.empty)) as TestImageInfo;
expect(h.value, equals(1));
// cache still has two entries in it: 2(7), 1(1)
final TestImageInfo i = await extractOneFrame(TestImageProvider(3, 9, image: await createTestImage()).resolve(ImageConfiguration.empty)) as TestImageInfo;
expect(i.value, equals(9));
// cache has three entries in it: 2(7), 1(1), 3(9)
final TestImageInfo j = await extractOneFrame(TestImageProvider(1, 10, image: await createTestImage()).resolve(ImageConfiguration.empty)) as TestImageInfo;
expect(j.value, equals(1));
// cache still has three entries in it: 2(7), 3(9), 1(1)
final TestImageInfo k = await extractOneFrame(TestImageProvider(4, 11, image: await createTestImage()).resolve(ImageConfiguration.empty)) as TestImageInfo;
expect(k.value, equals(11));
// cache has three entries: 3(9), 1(1), 4(11)
final TestImageInfo l = await extractOneFrame(TestImageProvider(1, 12, image: await createTestImage()).resolve(ImageConfiguration.empty)) as TestImageInfo;
expect(l.value, equals(1));
// cache has three entries: 3(9), 4(11), 1(1)
final TestImageInfo m = await extractOneFrame(TestImageProvider(2, 13, image: await createTestImage()).resolve(ImageConfiguration.empty)) as TestImageInfo;
expect(m.value, equals(13));
// cache has three entries: 4(11), 1(1), 2(13)
final TestImageInfo n = await extractOneFrame(TestImageProvider(3, 14, image: await createTestImage()).resolve(ImageConfiguration.empty)) as TestImageInfo;
expect(n.value, equals(14));
// cache has three entries: 1(1), 2(13), 3(14)
final TestImageInfo o = await extractOneFrame(TestImageProvider(4, 15, image: await createTestImage()).resolve(ImageConfiguration.empty)) as TestImageInfo;
expect(o.value, equals(15));
// cache has three entries: 2(13), 3(14), 4(15)
final TestImageInfo p = await extractOneFrame(TestImageProvider(1, 16, image: await createTestImage()).resolve(ImageConfiguration.empty)) as TestImageInfo;
expect(p.value, equals(16));
// cache has three entries: 3(14), 4(15), 1(16)
});
test('clear removes all images and resets cache size', () async {
final ui.Image testImage = await createTestImage(width: 8, height: 8);
expect(imageCache.currentSize, 0);
expect(imageCache.currentSizeBytes, 0);
await extractOneFrame(TestImageProvider(1, 1, image: testImage).resolve(ImageConfiguration.empty));
await extractOneFrame(TestImageProvider(2, 2, image: testImage).resolve(ImageConfiguration.empty));
expect(imageCache.currentSize, 2);
expect(imageCache.currentSizeBytes, 256 * 2);
imageCache.clear();
expect(imageCache.currentSize, 0);
expect(imageCache.currentSizeBytes, 0);
});
test('evicts individual images', () async {
final ui.Image testImage = await createTestImage(width: 8, height: 8);
await extractOneFrame(TestImageProvider(1, 1, image: testImage).resolve(ImageConfiguration.empty));
await extractOneFrame(TestImageProvider(2, 2, image: testImage).resolve(ImageConfiguration.empty));
expect(imageCache.currentSize, 2);
expect(imageCache.currentSizeBytes, 256 * 2);
expect(imageCache.evict(1), true);
expect(imageCache.currentSize, 1);
expect(imageCache.currentSizeBytes, 256);
});
test('Do not cache large images', () async {
final ui.Image testImage = await createTestImage(width: 8, height: 8);
imageCache.maximumSizeBytes = 1;
await extractOneFrame(TestImageProvider(1, 1, image: testImage).resolve(ImageConfiguration.empty));
expect(imageCache.currentSize, 0);
expect(imageCache.currentSizeBytes, 0);
expect(imageCache.maximumSizeBytes, 1);
});
test('Returns null if an error is caught resolving an image', () {
Future<ui.Codec> basicDecoder(ui.ImmutableBuffer bytes, {int? cacheWidth, int? cacheHeight, bool? allowUpscaling}) {
return PaintingBinding.instance.instantiateImageCodecFromBuffer(bytes, cacheWidth: cacheWidth, cacheHeight: cacheHeight, allowUpscaling: allowUpscaling ?? false);
}
final ErrorImageProvider errorImage = ErrorImageProvider();
expect(() => imageCache.putIfAbsent(errorImage, () => errorImage.loadBuffer(errorImage, basicDecoder)), throwsA(isA<Error>()));
bool caughtError = false;
final ImageStreamCompleter? result = imageCache.putIfAbsent(
errorImage,
() => errorImage.loadBuffer(errorImage, basicDecoder),
onError: (dynamic error, StackTrace? stackTrace) {
caughtError = true;
},
);
expect(result, null);
expect(caughtError, true);
});
test('already pending image is returned when it is put into the cache again', () async {
final ui.Image testImage = await createTestImage(width: 8, height: 8);
final TestImageStreamCompleter completer1 = TestImageStreamCompleter();
final TestImageStreamCompleter completer2 = TestImageStreamCompleter();
final TestImageStreamCompleter resultingCompleter1 = imageCache.putIfAbsent(testImage, () {
return completer1;
})! as TestImageStreamCompleter;
final TestImageStreamCompleter resultingCompleter2 = imageCache.putIfAbsent(testImage, () {
return completer2;
})! as TestImageStreamCompleter;
expect(resultingCompleter1, completer1);
expect(resultingCompleter2, completer1);
});
test('pending image is removed when cache is cleared', () async {
final ui.Image testImage = await createTestImage(width: 8, height: 8);
final TestImageStreamCompleter completer1 = TestImageStreamCompleter();
final TestImageStreamCompleter completer2 = TestImageStreamCompleter();
final TestImageStreamCompleter resultingCompleter1 = imageCache.putIfAbsent(testImage, () {
return completer1;
})! as TestImageStreamCompleter;
// Make the image seem live.
final ImageStreamListener listener = ImageStreamListener((_, __) {});
completer1.addListener(listener);
expect(imageCache.statusForKey(testImage).pending, true);
expect(imageCache.statusForKey(testImage).live, true);
imageCache.clear();
expect(imageCache.statusForKey(testImage).pending, false);
expect(imageCache.statusForKey(testImage).live, true);
imageCache.clearLiveImages();
expect(imageCache.statusForKey(testImage).pending, false);
expect(imageCache.statusForKey(testImage).live, false);
final TestImageStreamCompleter resultingCompleter2 = imageCache.putIfAbsent(testImage, () {
return completer2;
})! as TestImageStreamCompleter;
expect(resultingCompleter1, completer1);
expect(resultingCompleter2, completer2);
});
test('pending image is removed when image is evicted', () async {
final ui.Image testImage = await createTestImage(width: 8, height: 8);
final TestImageStreamCompleter completer1 = TestImageStreamCompleter();
final TestImageStreamCompleter completer2 = TestImageStreamCompleter();
final TestImageStreamCompleter resultingCompleter1 = imageCache.putIfAbsent(testImage, () {
return completer1;
})! as TestImageStreamCompleter;
imageCache.evict(testImage);
final TestImageStreamCompleter resultingCompleter2 = imageCache.putIfAbsent(testImage, () {
return completer2;
})! as TestImageStreamCompleter;
expect(resultingCompleter1, completer1);
expect(resultingCompleter2, completer2);
});
test("failed image can successfully be removed from the cache's pending images", () async {
final ui.Image testImage = await createTestImage(width: 8, height: 8);
FailingTestImageProvider(1, 1, image: testImage)
.resolve(ImageConfiguration.empty)
.addListener(ImageStreamListener(
(ImageInfo image, bool synchronousCall) {
fail('Image should not complete successfully');
},
onError: (dynamic exception, StackTrace? stackTrace) {
final bool evictionResult = imageCache.evict(1);
expect(evictionResult, isTrue);
},
));
// yield an event turn so that async work can complete.
await null;
});
test('containsKey - pending', () async {
final ui.Image testImage = await createTestImage(width: 8, height: 8);
final TestImageStreamCompleter completer1 = TestImageStreamCompleter();
final TestImageStreamCompleter resultingCompleter1 = imageCache.putIfAbsent(testImage, () {
return completer1;
})! as TestImageStreamCompleter;
expect(resultingCompleter1, completer1);
expect(imageCache.containsKey(testImage), true);
});
test('containsKey - completed', () async {
final ui.Image testImage = await createTestImage(width: 8, height: 8);
final TestImageStreamCompleter completer1 = TestImageStreamCompleter();
final TestImageStreamCompleter resultingCompleter1 = imageCache.putIfAbsent(testImage, () {
return completer1;
})! as TestImageStreamCompleter;
// Mark as complete
completer1.testSetImage(testImage);
expect(resultingCompleter1, completer1);
expect(imageCache.containsKey(testImage), true);
});
test('putIfAbsent updates LRU properties of a live image', () async {
imageCache.maximumSize = 1;
final ui.Image testImage = await createTestImage(width: 8, height: 8);
final ui.Image testImage2 = await createTestImage(width: 10, height: 10);
final TestImageStreamCompleter completer1 = TestImageStreamCompleter()..testSetImage(testImage);
final TestImageStreamCompleter completer2 = TestImageStreamCompleter()..testSetImage(testImage2);
completer1.addListener(ImageStreamListener((ImageInfo info, bool syncCall) {}));
final TestImageStreamCompleter resultingCompleter1 = imageCache.putIfAbsent(testImage, () {
return completer1;
})! as TestImageStreamCompleter;
expect(imageCache.statusForKey(testImage).pending, false);
expect(imageCache.statusForKey(testImage).keepAlive, true);
expect(imageCache.statusForKey(testImage).live, true);
expect(imageCache.statusForKey(testImage2).untracked, true);
final TestImageStreamCompleter resultingCompleter2 = imageCache.putIfAbsent(testImage2, () {
return completer2;
})! as TestImageStreamCompleter;
expect(imageCache.statusForKey(testImage).pending, false);
expect(imageCache.statusForKey(testImage).keepAlive, false); // evicted
expect(imageCache.statusForKey(testImage).live, true);
expect(imageCache.statusForKey(testImage2).pending, false);
expect(imageCache.statusForKey(testImage2).keepAlive, true); // took the LRU spot.
expect(imageCache.statusForKey(testImage2).live, false); // no listeners
expect(resultingCompleter1, completer1);
expect(resultingCompleter2, completer2);
});
test('Live image cache avoids leaks of unlistened streams', () async {
imageCache.maximumSize = 3;
TestImageProvider(1, 1, image: await createTestImage()).resolve(ImageConfiguration.empty);
TestImageProvider(2, 2, image: await createTestImage()).resolve(ImageConfiguration.empty);
TestImageProvider(3, 3, image: await createTestImage()).resolve(ImageConfiguration.empty);
TestImageProvider(4, 4, image: await createTestImage()).resolve(ImageConfiguration.empty);
TestImageProvider(5, 5, image: await createTestImage()).resolve(ImageConfiguration.empty);
TestImageProvider(6, 6, image: await createTestImage()).resolve(ImageConfiguration.empty);
// wait an event loop to let image resolution process.
await null;
expect(imageCache.currentSize, 3);
expect(imageCache.liveImageCount, 0);
});
test('Disabled image cache does not leak live images', () async {
imageCache.maximumSize = 0;
TestImageProvider(1, 1, image: await createTestImage()).resolve(ImageConfiguration.empty);
TestImageProvider(2, 2, image: await createTestImage()).resolve(ImageConfiguration.empty);
TestImageProvider(3, 3, image: await createTestImage()).resolve(ImageConfiguration.empty);
TestImageProvider(4, 4, image: await createTestImage()).resolve(ImageConfiguration.empty);
TestImageProvider(5, 5, image: await createTestImage()).resolve(ImageConfiguration.empty);
TestImageProvider(6, 6, image: await createTestImage()).resolve(ImageConfiguration.empty);
// wait an event loop to let image resolution process.
await null;
expect(imageCache.currentSize, 0);
expect(imageCache.liveImageCount, 0);
});
test('Clearing image cache does not leak live images', () async {
imageCache.maximumSize = 1;
final ui.Image testImage1 = await createTestImage(width: 8, height: 8);
final ui.Image testImage2 = await createTestImage(width: 10, height: 10);
final TestImageStreamCompleter completer1 = TestImageStreamCompleter();
final TestImageStreamCompleter completer2 = TestImageStreamCompleter()..testSetImage(testImage2);
imageCache.putIfAbsent(testImage1, () => completer1);
expect(imageCache.statusForKey(testImage1).pending, true);
expect(imageCache.statusForKey(testImage1).live, true);
imageCache.clear();
expect(imageCache.statusForKey(testImage1).pending, false);
expect(imageCache.statusForKey(testImage1).live, false);
completer1.testSetImage(testImage1);
expect(imageCache.statusForKey(testImage1).keepAlive, false);
expect(imageCache.statusForKey(testImage1).live, false);
imageCache.putIfAbsent(testImage2, () => completer2);
expect(imageCache.statusForKey(testImage1).tracked, false); // evicted
expect(imageCache.statusForKey(testImage2).tracked, true);
});
test('Evicting a pending image clears the live image by default', () async {
final ui.Image testImage = await createTestImage(width: 8, height: 8);
final TestImageStreamCompleter completer1 = TestImageStreamCompleter();
imageCache.putIfAbsent(testImage, () => completer1);
expect(imageCache.statusForKey(testImage).pending, true);
expect(imageCache.statusForKey(testImage).live, true);
expect(imageCache.statusForKey(testImage).keepAlive, false);
imageCache.evict(testImage);
expect(imageCache.statusForKey(testImage).untracked, true);
});
test('Evicting a pending image does clear the live image when includeLive is false and only cache listening', () async {
final ui.Image testImage = await createTestImage(width: 8, height: 8);
final TestImageStreamCompleter completer1 = TestImageStreamCompleter();
imageCache.putIfAbsent(testImage, () => completer1);
expect(imageCache.statusForKey(testImage).pending, true);
expect(imageCache.statusForKey(testImage).live, true);
expect(imageCache.statusForKey(testImage).keepAlive, false);
imageCache.evict(testImage, includeLive: false);
expect(imageCache.statusForKey(testImage).pending, false);
expect(imageCache.statusForKey(testImage).live, false);
expect(imageCache.statusForKey(testImage).keepAlive, false);
});
test('Evicting a pending image does clear the live image when includeLive is false and some other listener', () async {
final ui.Image testImage = await createTestImage(width: 8, height: 8);
final TestImageStreamCompleter completer1 = TestImageStreamCompleter();
imageCache.putIfAbsent(testImage, () => completer1);
expect(imageCache.statusForKey(testImage).pending, true);
expect(imageCache.statusForKey(testImage).live, true);
expect(imageCache.statusForKey(testImage).keepAlive, false);
completer1.addListener(ImageStreamListener((_, __) {}));
imageCache.evict(testImage, includeLive: false);
expect(imageCache.statusForKey(testImage).pending, false);
expect(imageCache.statusForKey(testImage).live, true);
expect(imageCache.statusForKey(testImage).keepAlive, false);
});
test('Evicting a completed image does clear the live image by default', () async {
final ui.Image testImage = await createTestImage(width: 8, height: 8);
final TestImageStreamCompleter completer1 = TestImageStreamCompleter()
..testSetImage(testImage)
..addListener(ImageStreamListener((ImageInfo info, bool syncCall) {}));
imageCache.putIfAbsent(testImage, () => completer1);
expect(imageCache.statusForKey(testImage).pending, false);
expect(imageCache.statusForKey(testImage).live, true);
expect(imageCache.statusForKey(testImage).keepAlive, true);
imageCache.evict(testImage);
expect(imageCache.statusForKey(testImage).untracked, true);
});
test('Evicting a completed image does not clear the live image when includeLive is set to false', () async {
final ui.Image testImage = await createTestImage(width: 8, height: 8);
final TestImageStreamCompleter completer1 = TestImageStreamCompleter()
..testSetImage(testImage)
..addListener(ImageStreamListener((ImageInfo info, bool syncCall) {}));
imageCache.putIfAbsent(testImage, () => completer1);
expect(imageCache.statusForKey(testImage).pending, false);
expect(imageCache.statusForKey(testImage).live, true);
expect(imageCache.statusForKey(testImage).keepAlive, true);
imageCache.evict(testImage, includeLive: false);
expect(imageCache.statusForKey(testImage).pending, false);
expect(imageCache.statusForKey(testImage).live, true);
expect(imageCache.statusForKey(testImage).keepAlive, false);
});
test('Clearing liveImages removes callbacks', () async {
final ui.Image testImage = await createTestImage(width: 8, height: 8);
final ImageStreamListener listener = ImageStreamListener((ImageInfo info, bool syncCall) {});
final TestImageStreamCompleter completer1 = TestImageStreamCompleter()
..testSetImage(testImage)
..addListener(listener);
final TestImageStreamCompleter completer2 = TestImageStreamCompleter()
..testSetImage(testImage)
..addListener(listener);
imageCache.putIfAbsent(testImage, () => completer1);
expect(imageCache.statusForKey(testImage).pending, false);
expect(imageCache.statusForKey(testImage).live, true);
expect(imageCache.statusForKey(testImage).keepAlive, true);
imageCache.clear();
imageCache.clearLiveImages();
expect(imageCache.statusForKey(testImage).pending, false);
expect(imageCache.statusForKey(testImage).live, false);
expect(imageCache.statusForKey(testImage).keepAlive, false);
imageCache.putIfAbsent(testImage, () => completer2);
expect(imageCache.statusForKey(testImage).pending, false);
expect(imageCache.statusForKey(testImage).live, true);
expect(imageCache.statusForKey(testImage).keepAlive, true);
completer1.removeListener(listener);
expect(imageCache.statusForKey(testImage).pending, false);
expect(imageCache.statusForKey(testImage).live, true);
expect(imageCache.statusForKey(testImage).keepAlive, true);
});
test('Live image gets size updated', () async {
// Add an image to the cache in pending state
// Complete it once it is in there as live
// Evict it but leave the live one.
// Add it again.
// If the live image did not track the size properly, the last line of
// this test will fail.
final ui.Image testImage = await createTestImage(width: 8, height: 8);
const int testImageSize = 8 * 8 * 4;
final ImageStreamListener listener = ImageStreamListener((ImageInfo info, bool syncCall) {});
final TestImageStreamCompleter completer1 = TestImageStreamCompleter()
..addListener(listener);
imageCache.putIfAbsent(testImage, () => completer1);
expect(imageCache.statusForKey(testImage).pending, true);
expect(imageCache.statusForKey(testImage).live, true);
expect(imageCache.statusForKey(testImage).keepAlive, false);
expect(imageCache.currentSizeBytes, 0);
completer1.testSetImage(testImage);
expect(imageCache.statusForKey(testImage).pending, false);
expect(imageCache.statusForKey(testImage).live, true);
expect(imageCache.statusForKey(testImage).keepAlive, true);
expect(imageCache.currentSizeBytes, testImageSize);
imageCache.evict(testImage, includeLive: false);
expect(imageCache.statusForKey(testImage).pending, false);
expect(imageCache.statusForKey(testImage).live, true);
expect(imageCache.statusForKey(testImage).keepAlive, false);
expect(imageCache.currentSizeBytes, 0);
imageCache.putIfAbsent(testImage, () => completer1);
expect(imageCache.statusForKey(testImage).pending, false);
expect(imageCache.statusForKey(testImage).live, true);
expect(imageCache.statusForKey(testImage).keepAlive, true);
expect(imageCache.currentSizeBytes, testImageSize);
});
test('Image is obtained and disposed of properly for cache', () async {
const int key = 1;
final ui.Image testImage = await createTestImage(width: 8, height: 8, cache: false);
expect(testImage.debugGetOpenHandleStackTraces()!.length, 1);
late ImageInfo imageInfo;
final ImageStreamListener listener = ImageStreamListener((ImageInfo info, bool syncCall) {
imageInfo = info;
});
final TestImageStreamCompleter completer = TestImageStreamCompleter();
completer.addListener(listener);
imageCache.putIfAbsent(key, () => completer);
expect(testImage.debugGetOpenHandleStackTraces()!.length, 1);
// This should cause keepAlive to be set to true.
completer.testSetImage(testImage);
expect(imageInfo, isNotNull);
// +1 ImageStreamCompleter
expect(testImage.debugGetOpenHandleStackTraces()!.length, 2);
completer.removeListener(listener);
// Force us to the end of the frame.
SchedulerBinding.instance.scheduleFrame();
await SchedulerBinding.instance.endOfFrame;
expect(testImage.debugGetOpenHandleStackTraces()!.length, 2);
expect(imageCache.evict(key), true);
// Force us to the end of the frame.
SchedulerBinding.instance.scheduleFrame();
await SchedulerBinding.instance.endOfFrame;
// -1 _CachedImage
// -1 ImageStreamCompleter
expect(testImage.debugGetOpenHandleStackTraces()!.length, 1);
imageInfo.dispose();
expect(testImage.debugGetOpenHandleStackTraces()!.length, 0);
}, skip: kIsWeb); // https://github.com/flutter/flutter/issues/87442
test('Image is obtained and disposed of properly for cache when listener is still active', () async {
const int key = 1;
final ui.Image testImage = await createTestImage(width: 8, height: 8, cache: false);
expect(testImage.debugGetOpenHandleStackTraces()!.length, 1);
late ImageInfo imageInfo;
final ImageStreamListener listener = ImageStreamListener((ImageInfo info, bool syncCall) {
imageInfo = info;
});
final TestImageStreamCompleter completer = TestImageStreamCompleter();
completer.addListener(listener);
imageCache.putIfAbsent(key, () => completer);
expect(testImage.debugGetOpenHandleStackTraces()!.length, 1);
// This should cause keepAlive to be set to true.
completer.testSetImage(testImage);
expect(imageInfo, isNotNull);
// Just our imageInfo and the completer.
expect(testImage.debugGetOpenHandleStackTraces()!.length, 2);
expect(imageCache.evict(key), true);
// Force us to the end of the frame.
SchedulerBinding.instance.scheduleFrame();
await SchedulerBinding.instance.endOfFrame;
// Live image still around since there's still a listener, and the listener
// should be holding a handle.
expect(testImage.debugGetOpenHandleStackTraces()!.length, 2);
completer.removeListener(listener);
expect(testImage.debugGetOpenHandleStackTraces()!.length, 1);
imageInfo.dispose();
expect(testImage.debugGetOpenHandleStackTraces()!.length, 0);
}, skip: kIsWeb); // https://github.com/flutter/flutter/issues/87442
test('clear does not leave pending images stuck', () async {
final ui.Image testImage = await createTestImage(width: 8, height: 8);
final TestImageStreamCompleter completer1 = TestImageStreamCompleter();
imageCache.putIfAbsent(testImage, () {
return completer1;
});
expect(imageCache.statusForKey(testImage).pending, true);
expect(imageCache.statusForKey(testImage).live, true);
expect(imageCache.statusForKey(testImage).keepAlive, false);
imageCache.clear();
// No one else is listening to the completer. It should not be considered
// live anymore.
expect(imageCache.statusForKey(testImage).pending, false);
expect(imageCache.statusForKey(testImage).live, false);
expect(imageCache.statusForKey(testImage).keepAlive, false);
});
}
| flutter/packages/flutter/test/painting/image_cache_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/painting/image_cache_test.dart",
"repo_id": "flutter",
"token_count": 8751
} | 699 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui;
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
class TestCanvas implements Canvas {
final List<Invocation> invocations = <Invocation>[];
@override
void noSuchMethod(Invocation invocation) {
invocations.add(invocation);
}
}
void main() {
late ui.Image image300x300;
late ui.Image image300x200;
setUpAll(() async {
image300x300 = await createTestImage(width: 300, height: 300, cache: false);
image300x200 = await createTestImage(width: 300, height: 200, cache: false);
});
setUp(() {
debugFlushLastFrameImageSizeInfo();
});
test('Cover and align', () async {
final TestCanvas canvas = TestCanvas();
paintImage(
canvas: canvas,
rect: const Rect.fromLTWH(50.0, 75.0, 200.0, 100.0),
image: image300x300,
fit: BoxFit.cover,
alignment: Alignment.centerLeft,
);
final Invocation command = canvas.invocations.firstWhere((Invocation invocation) {
return invocation.memberName == #drawImageRect;
});
expect(command, isNotNull);
expect(command.positionalArguments[0], equals(image300x300));
expect(command.positionalArguments[1], equals(const Rect.fromLTWH(0.0, 75.0, 300.0, 150.0)));
expect(command.positionalArguments[2], equals(const Rect.fromLTWH(50.0, 75.0, 200.0, 100.0)));
});
test('debugInvertOversizedImages', () async {
debugInvertOversizedImages = true;
expect(PaintingBinding.instance.platformDispatcher.views.any((ui. FlutterView view) => view.devicePixelRatio > 1.0), isTrue);
final FlutterExceptionHandler? oldFlutterError = FlutterError.onError;
final List<String> messages = <String>[];
FlutterError.onError = (FlutterErrorDetails details) {
messages.add(details.exceptionAsString());
};
final TestCanvas canvas = TestCanvas();
const Rect rect = Rect.fromLTWH(50.0, 50.0, 100.0, 50.0);
paintImage(
canvas: canvas,
rect: rect,
image: image300x300,
debugImageLabel: 'TestImage',
fit: BoxFit.fill,
);
final List<Invocation> commands = canvas.invocations
.skipWhile((Invocation invocation) => invocation.memberName != #saveLayer)
.take(4)
.toList();
expect(commands[0].positionalArguments[0], rect);
final Paint paint = commands[0].positionalArguments[1] as Paint;
expect(
paint.colorFilter,
const ColorFilter.matrix(<double>[
-1, 0, 0, 0, 255,
0, -1, 0, 0, 255,
0, 0, -1, 0, 255,
0, 0, 0, 1, 0,
]),
);
expect(commands[1].memberName, #translate);
expect(commands[1].positionalArguments[0], 0.0);
expect(commands[1].positionalArguments[1], 75.0);
expect(commands[2].memberName, #scale);
expect(commands[2].positionalArguments[0], 1.0);
expect(commands[2].positionalArguments[1], -1.0);
expect(commands[3].memberName, #translate);
expect(commands[3].positionalArguments[0], 0.0);
expect(commands[3].positionalArguments[1], -75.0);
expect(
messages.single,
'Image TestImage has a display size of 300×150 but a decode size of 300×300, which uses an additional 234KB (assuming a device pixel ratio of ${3.0}).\n\n'
'Consider resizing the asset ahead of time, supplying a cacheWidth parameter of 300, a cacheHeight parameter of 150, or using a ResizeImage.',
);
debugInvertOversizedImages = false;
FlutterError.onError = oldFlutterError;
});
test('debugInvertOversizedImages smaller than overhead allowance', () async {
debugInvertOversizedImages = true;
final FlutterExceptionHandler? oldFlutterError = FlutterError.onError;
final List<String> messages = <String>[];
FlutterError.onError = (FlutterErrorDetails details) {
messages.add(details.exceptionAsString());
};
try {
// Create a 290x290 sized image, which is ~24kb less than the allocated size,
// and below the default debugImageOverheadAllowance size of 128kb.
const Rect rect = Rect.fromLTWH(50.0, 50.0, 290.0, 290.0);
final TestCanvas canvas = TestCanvas();
paintImage(
canvas: canvas,
rect: rect,
image: image300x300,
debugImageLabel: 'TestImage',
fit: BoxFit.fill,
);
expect(messages, isEmpty);
} finally {
debugInvertOversizedImages = false;
FlutterError.onError = oldFlutterError;
}
});
test('centerSlice with scale ≠ 1', () async {
final TestCanvas canvas = TestCanvas();
paintImage(
canvas: canvas,
rect: const Rect.fromLTRB(10, 20, 430, 420),
image: image300x300,
scale: 2.0,
centerSlice: const Rect.fromLTRB(50, 40, 250, 260),
);
final Invocation command = canvas.invocations.firstWhere((Invocation invocation) {
return invocation.memberName == #drawImageNine;
});
expect(command, isNotNull);
expect(command.positionalArguments[0], equals(image300x300));
expect(command.positionalArguments[1], equals(const Rect.fromLTRB(100.0, 80.0, 500.0, 520.0)));
expect(command.positionalArguments[2], equals(const Rect.fromLTRB(20.0, 40.0, 860.0, 840.0)));
});
testWidgets('Reports Image painting', (WidgetTester tester) async {
late ImageSizeInfo imageSizeInfo;
int count = 0;
debugOnPaintImage = (ImageSizeInfo info) {
count += 1;
imageSizeInfo = info;
};
final TestCanvas canvas = TestCanvas();
paintImage(
canvas: canvas,
rect: const Rect.fromLTWH(50.0, 75.0, 200.0, 100.0),
image: image300x300,
debugImageLabel: 'test.png',
);
expect(count, 1);
expect(imageSizeInfo, isNotNull);
expect(imageSizeInfo.source, 'test.png');
expect(imageSizeInfo.imageSize, const Size(300, 300));
expect(imageSizeInfo.displaySize, const Size(200, 100) * tester.view.devicePixelRatio);
// Make sure that we don't report an identical image size info if we
// redraw in the next frame.
tester.binding.scheduleForcedFrame();
await tester.pump();
paintImage(
canvas: canvas,
rect: const Rect.fromLTWH(50.0, 75.0, 200.0, 100.0),
image: image300x300,
debugImageLabel: 'test.png',
);
expect(count, 1);
debugOnPaintImage = null;
});
testWidgets('Reports Image painting - change per frame', (WidgetTester tester) async {
late ImageSizeInfo imageSizeInfo;
int count = 0;
debugOnPaintImage = (ImageSizeInfo info) {
count += 1;
imageSizeInfo = info;
};
final TestCanvas canvas = TestCanvas();
paintImage(
canvas: canvas,
rect: const Rect.fromLTWH(50.0, 75.0, 200.0, 100.0),
image: image300x300,
debugImageLabel: 'test.png',
);
expect(count, 1);
expect(imageSizeInfo, isNotNull);
expect(imageSizeInfo.source, 'test.png');
expect(imageSizeInfo.imageSize, const Size(300, 300));
expect(imageSizeInfo.displaySize, const Size(200, 100) * tester.view.devicePixelRatio);
// Make sure that we don't report an identical image size info if we
// redraw in the next frame.
tester.binding.scheduleForcedFrame();
await tester.pump();
paintImage(
canvas: canvas,
rect: const Rect.fromLTWH(50.0, 75.0, 200.0, 150.0),
image: image300x300,
debugImageLabel: 'test.png',
);
expect(count, 2);
expect(imageSizeInfo, isNotNull);
expect(imageSizeInfo.source, 'test.png');
expect(imageSizeInfo.imageSize, const Size(300, 300));
expect(imageSizeInfo.displaySize, const Size(200, 150) * tester.view.devicePixelRatio);
debugOnPaintImage = null;
});
testWidgets('Reports Image painting - no debug label', (WidgetTester tester) async {
late ImageSizeInfo imageSizeInfo;
int count = 0;
debugOnPaintImage = (ImageSizeInfo info) {
count += 1;
imageSizeInfo = info;
};
final TestCanvas canvas = TestCanvas();
paintImage(
canvas: canvas,
rect: const Rect.fromLTWH(50.0, 75.0, 200.0, 100.0),
image: image300x200,
);
expect(count, 1);
expect(imageSizeInfo, isNotNull);
expect(imageSizeInfo.source, '<Unknown Image(300×200)>');
expect(imageSizeInfo.imageSize, const Size(300, 200));
expect(imageSizeInfo.displaySize, const Size(200, 100) * tester.view.devicePixelRatio);
debugOnPaintImage = null;
});
// See also the DecorationImage tests in: decoration_test.dart
}
| flutter/packages/flutter/test/painting/paint_image_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/painting/paint_image_test.dart",
"repo_id": "flutter",
"token_count": 3304
} | 700 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/physics.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('Friction simulation positive velocity', () {
final FrictionSimulation friction = FrictionSimulation(0.135, 100.0, 100.0);
expect(friction.x(0.0), moreOrLessEquals(100.0));
expect(friction.dx(0.0), moreOrLessEquals(100.0));
expect(friction.x(0.1), moreOrLessEquals(110.0, epsilon: 1.0));
expect(friction.x(0.5), moreOrLessEquals(131.0, epsilon: 1.0));
expect(friction.x(2.0), moreOrLessEquals(149.0, epsilon: 1.0));
expect(friction.finalX, moreOrLessEquals(149.0, epsilon: 1.0));
expect(friction.timeAtX(100.0), 0.0);
expect(friction.timeAtX(friction.x(0.1)), moreOrLessEquals(0.1));
expect(friction.timeAtX(friction.x(0.5)), moreOrLessEquals(0.5));
expect(friction.timeAtX(friction.x(2.0)), moreOrLessEquals(2.0));
expect(friction.timeAtX(-1.0), double.infinity);
expect(friction.timeAtX(200.0), double.infinity);
});
test('Friction simulation negative velocity', () {
final FrictionSimulation friction = FrictionSimulation(0.135, 100.0, -100.0);
expect(friction.x(0.0), moreOrLessEquals(100.0));
expect(friction.dx(0.0), moreOrLessEquals(-100.0));
expect(friction.x(0.1), moreOrLessEquals(91.0, epsilon: 1.0));
expect(friction.x(0.5), moreOrLessEquals(68.0, epsilon: 1.0));
expect(friction.x(2.0), moreOrLessEquals(51.0, epsilon: 1.0));
expect(friction.finalX, moreOrLessEquals(50, epsilon: 1.0));
expect(friction.timeAtX(100.0), 0.0);
expect(friction.timeAtX(friction.x(0.1)), moreOrLessEquals(0.1));
expect(friction.timeAtX(friction.x(0.5)), moreOrLessEquals(0.5));
expect(friction.timeAtX(friction.x(2.0)), moreOrLessEquals(2.0));
expect(friction.timeAtX(101.0), double.infinity);
expect(friction.timeAtX(40.0), double.infinity);
});
test('Friction simulation constant deceleration', () {
final FrictionSimulation friction = FrictionSimulation(0.135, 100.0, -100.0, constantDeceleration: 100);
expect(friction.x(0.0), moreOrLessEquals(100.0));
expect(friction.dx(0.0), moreOrLessEquals(-100.0));
expect(friction.x(0.1), moreOrLessEquals(91.0, epsilon: 1.0));
expect(friction.x(0.5), moreOrLessEquals(80.0, epsilon: 1.0));
expect(friction.x(2.0), moreOrLessEquals(80.0, epsilon: 1.0));
expect(friction.finalX, moreOrLessEquals(80.0, epsilon: 1.0));
expect(friction.timeAtX(100.0), 0.0);
expect(friction.timeAtX(friction.x(0.1)), moreOrLessEquals(0.1));
expect(friction.timeAtX(friction.x(0.2)), moreOrLessEquals(0.2));
expect(friction.timeAtX(friction.x(0.3)), moreOrLessEquals(0.3));
expect(friction.timeAtX(101.0), double.infinity);
expect(friction.timeAtX(40.0), double.infinity);
});
}
| flutter/packages/flutter/test/physics/friction_simulation_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/physics/friction_simulation_test.dart",
"repo_id": "flutter",
"token_count": 1240
} | 701 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import 'rendering_tester.dart';
void main() {
TestRenderingFlutterBinding.ensureInitialized();
test('RenderFractionallySizedBox constraints', () {
RenderBox root, leaf, test;
root = RenderPositionedBox(
child: RenderConstrainedBox(
additionalConstraints: BoxConstraints.tight(const Size(200.0, 200.0)),
child: test = RenderFractionallySizedOverflowBox(
widthFactor: 2.0,
heightFactor: 0.5,
child: leaf = RenderConstrainedBox(
additionalConstraints: const BoxConstraints.expand(),
),
),
),
);
layout(root);
expect(root.size.width, equals(800.0));
expect(root.size.height, equals(600.0));
expect(test.size.width, equals(200.0));
expect(test.size.height, equals(200.0));
expect(leaf.size.width, equals(400.0));
expect(leaf.size.height, equals(100.0));
});
test('BoxConstraints with NaN', () {
String result;
result = 'no exception';
try {
const BoxConstraints constraints = BoxConstraints(minWidth: double.nan, maxWidth: double.nan, minHeight: 2.0, maxHeight: double.nan);
assert(constraints.debugAssertIsValid());
} on FlutterError catch (e) {
result = '$e';
}
expect(result, equals(
'BoxConstraints has NaN values in minWidth, maxWidth, and maxHeight.\n'
'The offending constraints were:\n'
' BoxConstraints(NaN<=w<=NaN, 2.0<=h<=NaN; NOT NORMALIZED)',
));
result = 'no exception';
try {
const BoxConstraints constraints = BoxConstraints(minHeight: double.nan);
assert(constraints.debugAssertIsValid());
} on FlutterError catch (e) {
result = '$e';
}
expect(result, equals(
'BoxConstraints has a NaN value in minHeight.\n'
'The offending constraints were:\n'
' BoxConstraints(0.0<=w<=Infinity, NaN<=h<=Infinity; NOT NORMALIZED)',
));
result = 'no exception';
try {
const BoxConstraints constraints = BoxConstraints(minHeight: double.nan, maxWidth: 0.0/0.0);
assert(constraints.debugAssertIsValid());
} on FlutterError catch (e) {
result = '$e';
}
expect(result, equals(
'BoxConstraints has NaN values in maxWidth and minHeight.\n'
'The offending constraints were:\n'
' BoxConstraints(0.0<=w<=NaN, NaN<=h<=Infinity; NOT NORMALIZED)',
));
});
}
| flutter/packages/flutter/test/rendering/constraints_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/rendering/constraints_test.dart",
"repo_id": "flutter",
"token_count": 1059
} | 702 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import 'rendering_tester.dart';
void main() {
TestRenderingFlutterBinding.ensureInitialized();
test('LimitedBox: parent max size is unconstrained', () {
final RenderBox child = RenderConstrainedBox(
additionalConstraints: const BoxConstraints.tightFor(width: 300.0, height: 400.0),
);
final RenderBox parent = RenderConstrainedOverflowBox(
minWidth: 0.0,
maxWidth: double.infinity,
minHeight: 0.0,
maxHeight: double.infinity,
child: RenderLimitedBox(
maxWidth: 100.0,
maxHeight: 200.0,
child: child,
),
);
layout(parent);
expect(child.size.width, 100.0);
expect(child.size.height, 200.0);
expect(parent, hasAGoodToStringDeep);
expect(
parent.toStringDeep(minLevel: DiagnosticLevel.info),
equalsIgnoringHashCodes(
'RenderConstrainedOverflowBox#00000 NEEDS-PAINT NEEDS-COMPOSITING-BITS-UPDATE\n'
' │ parentData: <none>\n'
' │ constraints: BoxConstraints(w=800.0, h=600.0)\n'
' │ size: Size(800.0, 600.0)\n'
' │ alignment: Alignment.center\n'
' │ minWidth: 0.0\n'
' │ maxWidth: Infinity\n'
' │ minHeight: 0.0\n'
' │ maxHeight: Infinity\n'
' │ fit: max\n'
' │\n'
' └─child: RenderLimitedBox#00000 relayoutBoundary=up1 NEEDS-PAINT NEEDS-COMPOSITING-BITS-UPDATE\n'
' │ parentData: offset=Offset(350.0, 200.0) (can use size)\n'
' │ constraints: BoxConstraints(unconstrained)\n'
' │ size: Size(100.0, 200.0)\n'
' │ maxWidth: 100.0\n'
' │ maxHeight: 200.0\n'
' │\n'
' └─child: RenderConstrainedBox#00000 relayoutBoundary=up2 NEEDS-PAINT\n'
' parentData: <none> (can use size)\n'
' constraints: BoxConstraints(0.0<=w<=100.0, 0.0<=h<=200.0)\n'
' size: Size(100.0, 200.0)\n'
' additionalConstraints: BoxConstraints(w=300.0, h=400.0)\n',
),
);
});
test('LimitedBox: parent maxWidth is unconstrained', () {
final RenderBox child = RenderConstrainedBox(
additionalConstraints: const BoxConstraints.tightFor(width: 300.0, height: 400.0),
);
final RenderBox parent = RenderConstrainedOverflowBox(
minWidth: 0.0,
maxWidth: double.infinity,
minHeight: 500.0,
maxHeight: 500.0,
child: RenderLimitedBox(
maxWidth: 100.0,
maxHeight: 200.0,
child: child,
),
);
layout(parent);
expect(child.size.width, 100.0);
expect(child.size.height, 500.0);
});
test('LimitedBox: parent maxHeight is unconstrained', () {
final RenderBox child = RenderConstrainedBox(
additionalConstraints: const BoxConstraints.tightFor(width: 300.0, height: 400.0),
);
final RenderBox parent = RenderConstrainedOverflowBox(
minWidth: 500.0,
maxWidth: 500.0,
minHeight: 0.0,
maxHeight: double.infinity,
child: RenderLimitedBox(
maxWidth: 100.0,
maxHeight: 200.0,
child: child,
),
);
layout(parent);
expect(child.size.width, 500.0);
expect(child.size.height, 200.0);
});
test('LimitedBox: no child', () {
RenderBox box;
final RenderBox parent = RenderConstrainedOverflowBox(
minWidth: 10.0,
maxWidth: 500.0,
minHeight: 0.0,
maxHeight: double.infinity,
child: box = RenderLimitedBox(
maxWidth: 100.0,
maxHeight: 200.0,
),
);
layout(parent);
expect(box.size, const Size(10.0, 0.0));
expect(parent, hasAGoodToStringDeep);
expect(
parent.toStringDeep(minLevel: DiagnosticLevel.info),
equalsIgnoringHashCodes(
'RenderConstrainedOverflowBox#00000 NEEDS-PAINT NEEDS-COMPOSITING-BITS-UPDATE\n'
' │ parentData: <none>\n'
' │ constraints: BoxConstraints(w=800.0, h=600.0)\n'
' │ size: Size(800.0, 600.0)\n'
' │ alignment: Alignment.center\n'
' │ minWidth: 10.0\n'
' │ maxWidth: 500.0\n'
' │ minHeight: 0.0\n'
' │ maxHeight: Infinity\n'
' │ fit: max\n'
' │\n'
' └─child: RenderLimitedBox#00000 relayoutBoundary=up1 NEEDS-PAINT\n'
' parentData: offset=Offset(395.0, 300.0) (can use size)\n'
' constraints: BoxConstraints(10.0<=w<=500.0, 0.0<=h<=Infinity)\n'
' size: Size(10.0, 0.0)\n'
' maxWidth: 100.0\n'
' maxHeight: 200.0\n',
),
);
});
test('LimitedBox: no child use parent', () {
RenderBox box;
final RenderBox parent = RenderConstrainedOverflowBox(
minWidth: 10.0,
child: box = RenderLimitedBox(
maxWidth: 100.0,
maxHeight: 200.0,
),
);
layout(parent);
expect(box.size, const Size(10.0, 600.0));
expect(parent, hasAGoodToStringDeep);
expect(
parent.toStringDeep(minLevel: DiagnosticLevel.info),
equalsIgnoringHashCodes(
'RenderConstrainedOverflowBox#00000 NEEDS-PAINT NEEDS-COMPOSITING-BITS-UPDATE\n'
' │ parentData: <none>\n'
' │ constraints: BoxConstraints(w=800.0, h=600.0)\n'
' │ size: Size(800.0, 600.0)\n'
' │ alignment: Alignment.center\n'
' │ minWidth: 10.0\n'
' │ maxWidth: use parent maxWidth constraint\n'
' │ minHeight: use parent minHeight constraint\n'
' │ maxHeight: use parent maxHeight constraint\n'
' │ fit: max\n'
' │\n'
' └─child: RenderLimitedBox#00000 relayoutBoundary=up1 NEEDS-PAINT\n'
' parentData: offset=Offset(395.0, 0.0) (can use size)\n'
' constraints: BoxConstraints(10.0<=w<=800.0, h=600.0)\n'
' size: Size(10.0, 600.0)\n'
' maxWidth: 100.0\n'
' maxHeight: 200.0\n',
),
);
});
}
| flutter/packages/flutter/test/rendering/limited_box_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/rendering/limited_box_test.dart",
"repo_id": "flutter",
"token_count": 2828
} | 703 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui show BoxHeightStyle, BoxWidthStyle, Paragraph, TextBox;
import 'package:flutter/foundation.dart' show isCanvasKit, kIsWeb;
import 'package:flutter/gestures.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
import 'rendering_tester.dart';
const String _kText = "I polished up that handle so carefullee\nThat now I am the Ruler of the Queen's Navee!";
void _applyParentData(List<RenderBox> inlineRenderBoxes, InlineSpan span) {
int index = 0;
RenderBox? previousBox;
span.visitChildren((InlineSpan span) {
if (span is! WidgetSpan) {
return true;
}
final RenderBox box = inlineRenderBoxes[index];
box.parentData = TextParentData()
..span = span
..previousSibling = previousBox;
(previousBox?.parentData as TextParentData?)?.nextSibling = box;
index += 1;
previousBox = box;
return true;
});
}
// A subclass of RenderParagraph that returns an empty list in getBoxesForSelection
// for a given TextSelection.
// This is intended to simulate SkParagraph's implementation of Paragraph.getBoxesForRange,
// which may return an empty list in some situations where Libtxt would return a list
// containing an empty box.
class RenderParagraphWithEmptySelectionBoxList extends RenderParagraph {
RenderParagraphWithEmptySelectionBoxList(
super.text, {
required super.textDirection,
required this.emptyListSelection,
});
TextSelection emptyListSelection;
@override
List<ui.TextBox> getBoxesForSelection(
TextSelection selection, {
ui.BoxHeightStyle boxHeightStyle = ui.BoxHeightStyle.tight,
ui.BoxWidthStyle boxWidthStyle = ui.BoxWidthStyle.tight,
}) {
if (selection == emptyListSelection) {
return <ui.TextBox>[];
}
return super.getBoxesForSelection(
selection,
boxHeightStyle: boxHeightStyle,
boxWidthStyle: boxWidthStyle,
);
}
}
// A subclass of RenderParagraph that returns an empty list in getBoxesForSelection
// for a selection representing a WidgetSpan.
// This is intended to simulate how SkParagraph's implementation of Paragraph.getBoxesForRange
// can return an empty list for a WidgetSpan with empty dimensions.
class RenderParagraphWithEmptyBoxListForWidgetSpan extends RenderParagraph {
RenderParagraphWithEmptyBoxListForWidgetSpan(
super.text, {
required List<RenderBox> super.children,
required super.textDirection,
});
@override
List<ui.TextBox> getBoxesForSelection(
TextSelection selection, {
ui.BoxHeightStyle boxHeightStyle = ui.BoxHeightStyle.tight,
ui.BoxWidthStyle boxWidthStyle = ui.BoxWidthStyle.tight,
}) {
if (text.getSpanForPosition(selection.base) is WidgetSpan) {
return <ui.TextBox>[];
}
return super.getBoxesForSelection(
selection,
boxHeightStyle: boxHeightStyle,
boxWidthStyle: boxWidthStyle,
);
}
}
void main() {
TestRenderingFlutterBinding.ensureInitialized();
test('getOffsetForCaret control test', () {
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(text: _kText),
textDirection: TextDirection.ltr,
);
layout(paragraph);
const Rect caret = Rect.fromLTWH(0.0, 0.0, 2.0, 20.0);
final Offset offset5 = paragraph.getOffsetForCaret(const TextPosition(offset: 5), caret);
expect(offset5.dx, greaterThan(0.0));
final Offset offset25 = paragraph.getOffsetForCaret(const TextPosition(offset: 25), caret);
expect(offset25.dx, greaterThan(offset5.dx));
final Offset offset50 = paragraph.getOffsetForCaret(const TextPosition(offset: 50), caret);
expect(offset50.dy, greaterThan(offset5.dy));
});
test('getFullHeightForCaret control test', () {
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(text: _kText,style: TextStyle(fontSize: 10.0)),
textDirection: TextDirection.ltr,
);
layout(paragraph);
final double height5 = paragraph.getFullHeightForCaret(const TextPosition(offset: 5))!;
expect(height5, equals(10.0));
});
test('getPositionForOffset control test', () {
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(text: _kText),
textDirection: TextDirection.ltr,
);
layout(paragraph);
final TextPosition position20 = paragraph.getPositionForOffset(const Offset(20.0, 5.0));
expect(position20.offset, greaterThan(0.0));
final TextPosition position40 = paragraph.getPositionForOffset(const Offset(40.0, 5.0));
expect(position40.offset, greaterThan(position20.offset));
final TextPosition positionBelow = paragraph.getPositionForOffset(const Offset(5.0, 20.0));
expect(positionBelow.offset, greaterThan(position40.offset));
});
test('getBoxesForSelection control test', () {
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(text: _kText, style: TextStyle(fontSize: 10.0)),
textDirection: TextDirection.ltr,
);
layout(paragraph);
List<ui.TextBox> boxes = paragraph.getBoxesForSelection(
const TextSelection(baseOffset: 5, extentOffset: 25),
);
expect(boxes.length, equals(1));
boxes = paragraph.getBoxesForSelection(
const TextSelection(baseOffset: 25, extentOffset: 50),
);
expect(boxes.any((ui.TextBox box) => box.left == 250 && box.top == 0), isTrue);
expect(boxes.any((ui.TextBox box) => box.right == 100 && box.top == 10), isTrue);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/61016
test('getBoxesForSelection test with multiple TextSpans and lines', () {
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(
text: 'First ',
style: TextStyle(fontSize: 10.0),
children: <InlineSpan>[
TextSpan(text: 'smallsecond ', style: TextStyle(fontSize: 5.0)),
TextSpan(text: 'third fourth fifth'),
],
),
textDirection: TextDirection.ltr,
);
// Do layout with width chosen so that this splits as
// First smallsecond |
// third fourth |
// fifth|
// The corresponding line widths come out to be:
// 1st line: 120px wide: 6 chars * 10px plus 12 chars * 5px.
// 2nd line: 130px wide: 13 chars * 10px.
// 3rd line: 50px wide.
layout(paragraph, constraints: const BoxConstraints(maxWidth: 140.0));
final List<ui.TextBox> boxes = paragraph.getBoxesForSelection(
const TextSelection(baseOffset: 0, extentOffset: 36),
);
expect(boxes.length, equals(4));
// The widths of the boxes should match the calculations above.
// The heights should all be 10, except for the box for 'smallsecond ',
// which should have height 5, and be alphabetic baseline-aligned with
// 'First '. The test font specifies alphabetic baselines at 0.25em above
// the bottom extent, and 0.75em below the top, so the difference in top
// alignment becomes (10px * 0.75 - 5px * 0.75) = 3.75px.
// 'First ':
expect(boxes[0], const TextBox.fromLTRBD(0.0, 0.0, 60.0, 10.0, TextDirection.ltr));
// 'smallsecond ' in size 5:
expect(boxes[1], const TextBox.fromLTRBD(60.0, 3.75, 120.0, 8.75, TextDirection.ltr));
// 'third fourth ':
expect(boxes[2], const TextBox.fromLTRBD(0.0, 10.0, 130.0, 20.0, TextDirection.ltr));
// 'fifth':
expect(boxes[3], const TextBox.fromLTRBD(0.0, 20.0, 50.0, 30.0, TextDirection.ltr));
}, skip: kIsWeb && !isCanvasKit); // https://github.com/flutter/flutter/issues/61016
test('getBoxesForSelection test with boxHeightStyle and boxWidthStyle set to max', () {
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(
text: 'First ',
style: TextStyle(fontFamily: 'FlutterTest', fontSize: 10.0),
children: <InlineSpan>[
TextSpan(text: 'smallsecond ', style: TextStyle(fontSize: 8.0)),
TextSpan(text: 'third fourth fifth'),
],
),
textDirection: TextDirection.ltr,
);
// Do layout with width chosen so that this splits as
// First smallsecond |
// third fourth |
// fifth|
// The corresponding line widths come out to be:
// 1st line: 156px wide: 6 chars * 10px plus 12 chars * 8px.
// 2nd line: 130px wide: 13 chars * 10px.
// 3rd line: 50px wide.
layout(paragraph, constraints: const BoxConstraints(maxWidth: 160.0));
final List<ui.TextBox> boxes = paragraph.getBoxesForSelection(
const TextSelection(baseOffset: 0, extentOffset: 36),
boxHeightStyle: ui.BoxHeightStyle.max,
boxWidthStyle: ui.BoxWidthStyle.max,
);
expect(boxes.length, equals(5));
// 'First ':
expect(boxes[0], const TextBox.fromLTRBD(0.0, 0.0, 60.0, 10.0, TextDirection.ltr));
// 'smallsecond ' in size 8, but on same line as previous box, so height remains 10:
expect(boxes[1], const TextBox.fromLTRBD(60.0, 0.0, 156.0, 10.0, TextDirection.ltr));
// 'third fourth ':
expect(boxes[2], const TextBox.fromLTRBD(0.0, 10.0, 130.0, 20.0, TextDirection.ltr));
// extra box added to extend width, as per definition of ui.BoxWidthStyle.max:
expect(boxes[3], const TextBox.fromLTRBD(130.0, 10.0, 156.0, 20.0, TextDirection.ltr));
// 'fifth':
expect(boxes[4], const TextBox.fromLTRBD(0.0, 20.0, 50.0, 30.0, TextDirection.ltr));
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/61016
test('getWordBoundary control test', () {
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(text: _kText),
textDirection: TextDirection.ltr,
);
layout(paragraph);
final TextRange range5 = paragraph.getWordBoundary(const TextPosition(offset: 5));
expect(range5.textInside(_kText), equals('polished'));
final TextRange range50 = paragraph.getWordBoundary(const TextPosition(offset: 50));
expect(range50.textInside(_kText), equals(' '));
final TextRange range85 = paragraph.getWordBoundary(const TextPosition(offset: 75));
expect(range85.textInside(_kText), equals("Queen's"));
});
test('overflow test', () {
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(
text: 'This\n' // 4 characters * 10px font size = 40px width on the first line
'is a wrapping test. It should wrap at manual newlines, and if softWrap is true, also at spaces.',
style: TextStyle(fontSize: 10.0),
),
textDirection: TextDirection.ltr,
maxLines: 1,
);
void relayoutWith({
int? maxLines,
required bool softWrap,
required TextOverflow overflow,
}) {
paragraph
..maxLines = maxLines
..softWrap = softWrap
..overflow = overflow;
pumpFrame();
}
// Lay out in a narrow box to force wrapping.
layout(paragraph, constraints: const BoxConstraints(maxWidth: 50.0)); // enough to fit "This" but not "This is"
final double lineHeight = paragraph.size.height;
relayoutWith(maxLines: 3, softWrap: true, overflow: TextOverflow.clip);
expect(paragraph.size.height, equals(3 * lineHeight));
relayoutWith(softWrap: true, overflow: TextOverflow.clip);
expect(paragraph.size.height, greaterThan(5 * lineHeight));
// Try again with ellipsis overflow. We can't test that the ellipsis are
// drawn, but we can test the sizing.
relayoutWith(maxLines: 1, softWrap: true, overflow: TextOverflow.ellipsis);
expect(paragraph.size.height, equals(lineHeight));
relayoutWith(maxLines: 3, softWrap: true, overflow: TextOverflow.ellipsis);
expect(paragraph.size.height, equals(3 * lineHeight));
// This is the one weird case. If maxLines is null, we would expect to allow
// infinite wrapping. However, if we did, we'd never know when to append an
// ellipsis, so this really means "append ellipsis as soon as we exceed the
// width".
relayoutWith(softWrap: true, overflow: TextOverflow.ellipsis);
expect(paragraph.size.height, equals(2 * lineHeight));
// Now with no soft wrapping.
relayoutWith(maxLines: 1, softWrap: false, overflow: TextOverflow.clip);
expect(paragraph.size.height, equals(lineHeight));
relayoutWith(maxLines: 3, softWrap: false, overflow: TextOverflow.clip);
expect(paragraph.size.height, equals(2 * lineHeight));
relayoutWith(softWrap: false, overflow: TextOverflow.clip);
expect(paragraph.size.height, equals(2 * lineHeight));
relayoutWith(maxLines: 1, softWrap: false, overflow: TextOverflow.ellipsis);
expect(paragraph.size.height, equals(lineHeight));
relayoutWith(maxLines: 3, softWrap: false, overflow: TextOverflow.ellipsis);
expect(paragraph.size.height, equals(3 * lineHeight));
relayoutWith(softWrap: false, overflow: TextOverflow.ellipsis);
expect(paragraph.size.height, equals(2 * lineHeight));
// Test presence of the fade effect.
relayoutWith(maxLines: 3, softWrap: true, overflow: TextOverflow.fade);
expect(paragraph.debugHasOverflowShader, isTrue);
// Change back to ellipsis and check that the fade shader is cleared.
relayoutWith(maxLines: 3, softWrap: true, overflow: TextOverflow.ellipsis);
expect(paragraph.debugHasOverflowShader, isFalse);
relayoutWith(maxLines: 100, softWrap: true, overflow: TextOverflow.fade);
expect(paragraph.debugHasOverflowShader, isFalse);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/61018
test('maxLines', () {
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(
text: "How do you write like you're running out of time? Write day and night like you're running out of time?",
// 0123456789 0123456789 012 345 0123456 012345 01234 012345678 012345678 0123 012 345 0123456 012345 01234
// 0 1 2 3 4 5 6 7 8 9 10 11 12
style: TextStyle(fontSize: 10.0),
),
textDirection: TextDirection.ltr,
);
layout(paragraph, constraints: const BoxConstraints(maxWidth: 100.0));
void layoutAt(int? maxLines) {
paragraph.maxLines = maxLines;
pumpFrame();
}
layoutAt(null);
expect(paragraph.size.height, 130.0);
layoutAt(1);
expect(paragraph.size.height, 10.0);
layoutAt(2);
expect(paragraph.size.height, 20.0);
layoutAt(3);
expect(paragraph.size.height, 30.0);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/61018
test('textAlign triggers TextPainter relayout in the paint method', () {
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(text: 'A', style: TextStyle(fontSize: 10.0)),
textDirection: TextDirection.ltr,
textAlign: TextAlign.left,
);
Rect getRectForA() => paragraph.getBoxesForSelection(const TextSelection(baseOffset: 0, extentOffset: 1)).single.toRect();
layout(paragraph, constraints: const BoxConstraints.tightFor(width: 100.0));
expect(getRectForA(), const Rect.fromLTWH(0, 0, 10, 10));
paragraph.textAlign = TextAlign.right;
expect(paragraph.debugNeedsLayout, isFalse);
expect(paragraph.debugNeedsPaint, isTrue);
paragraph.paint(MockPaintingContext(), Offset.zero);
expect(getRectForA(), const Rect.fromLTWH(90, 0, 10, 10));
});
group('didExceedMaxLines', () {
RenderParagraph createRenderParagraph({
int? maxLines,
TextOverflow overflow = TextOverflow.clip,
}) {
return RenderParagraph(
const TextSpan(
text: 'Here is a long text, maybe exceed maxlines',
style: TextStyle(fontSize: 10.0),
),
textDirection: TextDirection.ltr,
overflow: overflow,
maxLines: maxLines,
);
}
test('none limited', () {
final RenderParagraph paragraph = createRenderParagraph();
layout(paragraph, constraints: const BoxConstraints(maxWidth: 100.0));
expect(paragraph.didExceedMaxLines, false);
});
test('limited by maxLines', () {
final RenderParagraph paragraph = createRenderParagraph(maxLines: 1);
layout(paragraph, constraints: const BoxConstraints(maxWidth: 100.0));
expect(paragraph.didExceedMaxLines, true);
});
test('limited by ellipsis', () {
final RenderParagraph paragraph = createRenderParagraph(overflow: TextOverflow.ellipsis);
layout(paragraph, constraints: const BoxConstraints(maxWidth: 100.0));
expect(paragraph.didExceedMaxLines, true);
});
});
test('changing color does not do layout', () {
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(
text: 'Hello',
style: TextStyle(color: Color(0xFF000000)),
),
textDirection: TextDirection.ltr,
);
layout(paragraph, constraints: const BoxConstraints(maxWidth: 100.0), phase: EnginePhase.paint);
expect(paragraph.debugNeedsLayout, isFalse);
expect(paragraph.debugNeedsPaint, isFalse);
paragraph.text = const TextSpan(
text: 'Hello World',
style: TextStyle(color: Color(0xFF000000)),
);
expect(paragraph.debugNeedsLayout, isTrue);
expect(paragraph.debugNeedsPaint, isFalse);
pumpFrame(phase: EnginePhase.paint);
expect(paragraph.debugNeedsLayout, isFalse);
expect(paragraph.debugNeedsPaint, isFalse);
paragraph.text = const TextSpan(
text: 'Hello World',
style: TextStyle(color: Color(0xFFFFFFFF)),
);
expect(paragraph.debugNeedsLayout, isFalse);
expect(paragraph.debugNeedsPaint, isTrue);
pumpFrame(phase: EnginePhase.paint);
expect(paragraph.debugNeedsLayout, isFalse);
expect(paragraph.debugNeedsPaint, isFalse);
});
test('nested TextSpans in paragraph handle linear textScaler correctly.', () {
const TextSpan testSpan = TextSpan(
text: 'a',
style: TextStyle(
fontSize: 10.0,
),
children: <TextSpan>[
TextSpan(
text: 'b',
children: <TextSpan>[
TextSpan(text: 'c'),
],
style: TextStyle(
fontSize: 20.0,
),
),
TextSpan(
text: 'd',
),
],
);
final RenderParagraph paragraph = RenderParagraph(
testSpan,
textDirection: TextDirection.ltr,
textScaler: const TextScaler.linear(1.3),
);
paragraph.layout(const BoxConstraints());
expect(paragraph.size.width, 78.0);
expect(paragraph.size.height, 26.0);
final int length = testSpan.toPlainText().length;
// Test the sizes of nested spans.
final List<ui.TextBox> boxes = <ui.TextBox>[
for (int i = 0; i < length; ++i)
...paragraph.getBoxesForSelection(
TextSelection(baseOffset: i, extentOffset: i + 1),
),
];
expect(boxes, hasLength(4));
expect(boxes[0].toRect().width, 13.0);
expect(boxes[0].toRect().height, 13.0);
expect(boxes[1].toRect().width, 26.0);
expect(boxes[1].toRect().height, 26.0);
expect(boxes[2].toRect().width, 26.0);
expect(boxes[2].toRect().height, 26.0);
expect(boxes[3].toRect().width, 13.0);
expect(boxes[3].toRect().height, 13.0);
});
test('toStringDeep', () {
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(text: _kText),
textDirection: TextDirection.ltr,
locale: const Locale('ja', 'JP'),
);
expect(paragraph, hasAGoodToStringDeep);
expect(
paragraph.toStringDeep(minLevel: DiagnosticLevel.info),
equalsIgnoringHashCodes(
'RenderParagraph#00000 NEEDS-LAYOUT NEEDS-PAINT DETACHED\n'
' │ parentData: MISSING\n'
' │ constraints: MISSING\n'
' │ size: MISSING\n'
' │ textAlign: start\n'
' │ textDirection: ltr\n'
' │ softWrap: wrapping at box width\n'
' │ overflow: clip\n'
' │ locale: ja_JP\n'
' │ maxLines: unlimited\n'
' ╘═╦══ text ═══\n'
' ║ TextSpan:\n'
' ║ "I polished up that handle so carefullee\n'
' ║ That now I am the Ruler of the Queen\'s Navee!"\n'
' ╚═══════════\n',
),
);
});
test('locale setter', () {
// Regression test for https://github.com/flutter/flutter/issues/18175
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(text: _kText),
locale: const Locale('zh', 'HK'),
textDirection: TextDirection.ltr,
);
expect(paragraph.locale, const Locale('zh', 'HK'));
paragraph.locale = const Locale('ja', 'JP');
expect(paragraph.locale, const Locale('ja', 'JP'));
});
test('inline widgets test', () {
const TextSpan text = TextSpan(
text: 'a',
style: TextStyle(fontSize: 10.0),
children: <InlineSpan>[
WidgetSpan(child: SizedBox(width: 21, height: 21)),
WidgetSpan(child: SizedBox(width: 21, height: 21)),
TextSpan(text: 'a'),
WidgetSpan(child: SizedBox(width: 21, height: 21)),
],
);
// Fake the render boxes that correspond to the WidgetSpans. We use
// RenderParagraph to reduce dependencies this test has.
final List<RenderBox> renderBoxes = <RenderBox>[
RenderParagraph(const TextSpan(text: 'b'), textDirection: TextDirection.ltr),
RenderParagraph(const TextSpan(text: 'b'), textDirection: TextDirection.ltr),
RenderParagraph(const TextSpan(text: 'b'), textDirection: TextDirection.ltr),
];
final RenderParagraph paragraph = RenderParagraph(
text,
textDirection: TextDirection.ltr,
children: renderBoxes,
);
_applyParentData(renderBoxes, text);
layout(paragraph, constraints: const BoxConstraints(maxWidth: 100.0));
final List<ui.TextBox> boxes = paragraph.getBoxesForSelection(
const TextSelection(baseOffset: 0, extentOffset: 8),
);
expect(boxes.length, equals(5));
expect(boxes[0], const TextBox.fromLTRBD(0.0, 4.0, 10.0, 14.0, TextDirection.ltr));
expect(boxes[1], const TextBox.fromLTRBD(10.0, 0.0, 24.0, 14.0, TextDirection.ltr));
expect(boxes[2], const TextBox.fromLTRBD(24.0, 0.0, 38.0, 14.0, TextDirection.ltr));
expect(boxes[3], const TextBox.fromLTRBD(38.0, 4.0, 48.0, 14.0, TextDirection.ltr));
expect(boxes[4], const TextBox.fromLTRBD(48.0, 0.0, 62.0, 14.0, TextDirection.ltr));
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/61020
test('getBoxesForSelection with boxHeightStyle for inline widgets', () {
const TextSpan text = TextSpan(
text: 'a',
style: TextStyle(fontSize: 10.0),
children: <InlineSpan>[
WidgetSpan(child: SizedBox(width: 21, height: 21)),
WidgetSpan(child: SizedBox(width: 21, height: 21)),
TextSpan(text: 'a'),
WidgetSpan(child: SizedBox(width: 21, height: 21)),
],
);
// Fake the render boxes that correspond to the WidgetSpans. We use
// RenderParagraph to reduce the dependencies this test has. The dimensions
// of these get used in place of the widths and heights specified in the
// SizedBoxes above: each comes out as (w,h) = (14,14).
final List<RenderBox> renderBoxes = <RenderBox>[
RenderParagraph(const TextSpan(text: 'b'), textDirection: TextDirection.ltr),
RenderParagraph(const TextSpan(text: 'b'), textDirection: TextDirection.ltr),
RenderParagraph(const TextSpan(text: 'b'), textDirection: TextDirection.ltr),
];
final RenderParagraph paragraph = RenderParagraph(
text,
textDirection: TextDirection.ltr,
children: renderBoxes,
);
_applyParentData(renderBoxes, text);
layout(paragraph, constraints: const BoxConstraints(maxWidth: 100.0));
final List<ui.TextBox> boxes = paragraph.getBoxesForSelection(
const TextSelection(baseOffset: 0, extentOffset: 8),
boxHeightStyle: ui.BoxHeightStyle.max,
);
expect(boxes.length, equals(5));
expect(boxes[0], const TextBox.fromLTRBD(0.0, 0.0, 10.0, 14.0, TextDirection.ltr));
expect(boxes[1], const TextBox.fromLTRBD(10.0, 0.0, 24.0, 14.0, TextDirection.ltr));
expect(boxes[2], const TextBox.fromLTRBD(24.0, 0.0, 38.0, 14.0, TextDirection.ltr));
expect(boxes[3], const TextBox.fromLTRBD(38.0, 0.0, 48.0, 14.0, TextDirection.ltr));
expect(boxes[4], const TextBox.fromLTRBD(48.0, 0.0, 62.0, 14.0, TextDirection.ltr));
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/61020
test('inline widgets multiline test', () {
const TextSpan text = TextSpan(
text: 'a',
style: TextStyle(fontSize: 10.0),
children: <InlineSpan>[
WidgetSpan(child: SizedBox(width: 21, height: 21)),
WidgetSpan(child: SizedBox(width: 21, height: 21)),
TextSpan(text: 'a'),
WidgetSpan(child: SizedBox(width: 21, height: 21)),
WidgetSpan(child: SizedBox(width: 21, height: 21)),
WidgetSpan(child: SizedBox(width: 21, height: 21)),
WidgetSpan(child: SizedBox(width: 21, height: 21)),
WidgetSpan(child: SizedBox(width: 21, height: 21)),
],
);
// Fake the render boxes that correspond to the WidgetSpans. We use
// RenderParagraph to reduce dependencies this test has.
final List<RenderBox> renderBoxes = <RenderBox>[
RenderParagraph(const TextSpan(text: 'b'), textDirection: TextDirection.ltr),
RenderParagraph(const TextSpan(text: 'b'), textDirection: TextDirection.ltr),
RenderParagraph(const TextSpan(text: 'b'), textDirection: TextDirection.ltr),
RenderParagraph(const TextSpan(text: 'b'), textDirection: TextDirection.ltr),
RenderParagraph(const TextSpan(text: 'b'), textDirection: TextDirection.ltr),
RenderParagraph(const TextSpan(text: 'b'), textDirection: TextDirection.ltr),
RenderParagraph(const TextSpan(text: 'b'), textDirection: TextDirection.ltr),
];
final RenderParagraph paragraph = RenderParagraph(
text,
textDirection: TextDirection.ltr,
children: renderBoxes,
);
_applyParentData(renderBoxes, text);
layout(paragraph, constraints: const BoxConstraints(maxWidth: 50.0));
final List<ui.TextBox> boxes = paragraph.getBoxesForSelection(
const TextSelection(baseOffset: 0, extentOffset: 12),
);
expect(boxes.length, equals(9));
expect(boxes[0], const TextBox.fromLTRBD(0.0, 4.0, 10.0, 14.0, TextDirection.ltr));
expect(boxes[1], const TextBox.fromLTRBD(10.0, 0.0, 24.0, 14.0, TextDirection.ltr));
expect(boxes[2], const TextBox.fromLTRBD(24.0, 0.0, 38.0, 14.0, TextDirection.ltr));
expect(boxes[3], const TextBox.fromLTRBD(38.0, 4.0, 48.0, 14.0, TextDirection.ltr));
// Wraps
expect(boxes[4], const TextBox.fromLTRBD(0.0, 14.0, 14.0, 28.0 , TextDirection.ltr));
expect(boxes[5], const TextBox.fromLTRBD(14.0, 14.0, 28.0, 28.0, TextDirection.ltr));
expect(boxes[6], const TextBox.fromLTRBD(28.0, 14.0, 42.0, 28.0, TextDirection.ltr));
// Wraps
expect(boxes[7], const TextBox.fromLTRBD(0.0, 28.0, 14.0, 42.0, TextDirection.ltr));
expect(boxes[8], const TextBox.fromLTRBD(14.0, 28.0, 28.0, 42.0 , TextDirection.ltr));
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/61020
test('Does not include the semantics node of truncated rendering children', () {
// Regression test for https://github.com/flutter/flutter/issues/88180
const double screenWidth = 100;
const String sentence = 'truncated';
final List<RenderBox> renderBoxes = <RenderBox>[
RenderParagraph(
const TextSpan(text: sentence), textDirection: TextDirection.ltr),
];
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(
text: 'a long line to be truncated.',
children: <InlineSpan>[
WidgetSpan(child: Text(sentence)),
],
),
overflow: TextOverflow.ellipsis,
children: renderBoxes,
textDirection: TextDirection.ltr,
);
_applyParentData(renderBoxes, paragraph.text);
layout(paragraph, constraints: const BoxConstraints(maxWidth: screenWidth));
final SemanticsNode result = SemanticsNode();
final SemanticsNode truncatedChild = SemanticsNode();
truncatedChild.tags = <SemanticsTag>{const PlaceholderSpanIndexSemanticsTag(0)};
paragraph.assembleSemanticsNode(result, SemanticsConfiguration(), <SemanticsNode>[truncatedChild]);
// It should only contain the semantics node of the TextSpan.
expect(result.childrenCount, 1);
result.visitChildren((SemanticsNode node) {
expect(node != truncatedChild, isTrue);
return true;
});
});
test('Supports gesture recognizer semantics', () {
final RenderParagraph paragraph = RenderParagraph(
TextSpan(text: _kText, children: <InlineSpan>[
TextSpan(text: 'one', recognizer: TapGestureRecognizer()..onTap = () {}),
TextSpan(text: 'two', recognizer: LongPressGestureRecognizer()..onLongPress = () {}),
TextSpan(text: 'three', recognizer: DoubleTapGestureRecognizer()..onDoubleTap = () {}),
]),
textDirection: TextDirection.rtl,
);
layout(paragraph);
final SemanticsNode node = SemanticsNode();
paragraph.assembleSemanticsNode(node, SemanticsConfiguration(), <SemanticsNode>[]);
final List<SemanticsNode> children = <SemanticsNode>[];
node.visitChildren((SemanticsNode child) {
children.add(child);
return true;
});
expect(children.length, 4);
expect(children[0].getSemanticsData().actions, 0);
expect(children[1].getSemanticsData().hasAction(SemanticsAction.tap), true);
expect(children[2].getSemanticsData().hasAction(SemanticsAction.longPress), true);
expect(children[3].getSemanticsData().hasAction(SemanticsAction.tap), true);
});
test('Supports empty text span with spell out', () {
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(text: '', spellOut: true),
textDirection: TextDirection.rtl,
);
layout(paragraph);
final SemanticsNode node = SemanticsNode();
paragraph.assembleSemanticsNode(node, SemanticsConfiguration(), <SemanticsNode>[]);
expect(node.attributedLabel.string, '');
expect(node.attributedLabel.attributes.length, 0);
});
test('Asserts on unsupported gesture recognizer', () {
final RenderParagraph paragraph = RenderParagraph(
TextSpan(text: _kText, children: <InlineSpan>[
TextSpan(text: 'three', recognizer: MultiTapGestureRecognizer()..onTap = (int id) {}),
]),
textDirection: TextDirection.rtl,
);
layout(paragraph);
bool failed = false;
try {
paragraph.assembleSemanticsNode(SemanticsNode(), SemanticsConfiguration(), <SemanticsNode>[]);
} on AssertionError catch (e) {
failed = true;
expect(e.message, 'MultiTapGestureRecognizer is not supported.');
}
expect(failed, true);
});
test('assembleSemanticsNode handles text spans that do not yield selection boxes', () {
final RenderParagraph paragraph = RenderParagraphWithEmptySelectionBoxList(
TextSpan(text: '', children: <InlineSpan>[
TextSpan(text: 'A', recognizer: TapGestureRecognizer()..onTap = () {}),
TextSpan(text: 'B', recognizer: TapGestureRecognizer()..onTap = () {}),
TextSpan(text: 'C', recognizer: TapGestureRecognizer()..onTap = () {}),
]),
textDirection: TextDirection.rtl,
emptyListSelection: const TextSelection(baseOffset: 0, extentOffset: 1),
);
layout(paragraph);
final SemanticsNode node = SemanticsNode();
paragraph.assembleSemanticsNode(node, SemanticsConfiguration(), <SemanticsNode>[]);
expect(node.childrenCount, 2);
});
test('assembleSemanticsNode handles empty WidgetSpans that do not yield selection boxes', () {
final TextSpan text = TextSpan(text: '', children: <InlineSpan>[
TextSpan(text: 'A', recognizer: TapGestureRecognizer()..onTap = () {}),
const WidgetSpan(child: SizedBox.shrink()),
TextSpan(text: 'C', recognizer: TapGestureRecognizer()..onTap = () {}),
]);
final List<RenderBox> renderBoxes = <RenderBox>[
RenderParagraph(const TextSpan(text: 'b'), textDirection: TextDirection.ltr),
];
final RenderParagraph paragraph = RenderParagraphWithEmptyBoxListForWidgetSpan(
text,
children: renderBoxes,
textDirection: TextDirection.ltr,
);
_applyParentData(renderBoxes, paragraph.text);
layout(paragraph);
final SemanticsNode node = SemanticsNode();
paragraph.assembleSemanticsNode(node, SemanticsConfiguration(), <SemanticsNode>[]);
expect(node.childrenCount, 2);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/61020
test('Basic TextSpan Hit testing', () {
final TextSpan textSpanA = TextSpan(text: 'A' * 10);
const TextSpan textSpanBC = TextSpan(text: 'BC', style: TextStyle(letterSpacing: 26.0));
final TextSpan text = TextSpan(
style: const TextStyle(fontSize: 10.0),
children: <InlineSpan>[textSpanA, textSpanBC],
);
final RenderParagraph paragraph = RenderParagraph(text, textDirection: TextDirection.ltr);
layout(paragraph, constraints: const BoxConstraints.tightFor(width: 100.0));
BoxHitTestResult result;
// Hit-testing the first line
// First A
expect(paragraph.hitTest(result = BoxHitTestResult(), position: const Offset(5.0, 5.0)), isTrue);
expect(result.path.map((HitTestEntry<HitTestTarget> entry) => entry.target).whereType<TextSpan>(), <TextSpan>[textSpanA]);
// The last A.
expect(paragraph.hitTest(result = BoxHitTestResult(), position: const Offset(95.0, 5.0)), isTrue);
expect(result.path.map((HitTestEntry<HitTestTarget> entry) => entry.target).whereType<TextSpan>(), <TextSpan>[textSpanA]);
// Far away from the line.
expect(paragraph.hitTest(result = BoxHitTestResult(), position: const Offset(200.0, 5.0)), isFalse);
expect(result.path.map((HitTestEntry<HitTestTarget> entry) => entry.target).whereType<TextSpan>(), <TextSpan>[]);
// Hit-testing the second line
// Tapping on B (startX = letter-spacing / 2 = 13.0).
expect(paragraph.hitTest(result = BoxHitTestResult(), position: const Offset(18.0, 15.0)), isTrue);
expect(result.path.map((HitTestEntry<HitTestTarget> entry) => entry.target).whereType<TextSpan>(), <TextSpan>[textSpanBC]);
// Between B and C, with large letter-spacing.
expect(paragraph.hitTest(result = BoxHitTestResult(), position: const Offset(31.0, 15.0)), isTrue);
expect(result.path.map((HitTestEntry<HitTestTarget> entry) => entry.target).whereType<TextSpan>(), <TextSpan>[textSpanBC]);
// On C.
expect(paragraph.hitTest(result = BoxHitTestResult(), position: const Offset(54.0, 15.0)), isTrue);
expect(result.path.map((HitTestEntry<HitTestTarget> entry) => entry.target).whereType<TextSpan>(), <TextSpan>[textSpanBC]);
// After C.
expect(paragraph.hitTest(result = BoxHitTestResult(), position: const Offset(100.0, 15.0)), isFalse);
expect(result.path.map((HitTestEntry<HitTestTarget> entry) => entry.target).whereType<TextSpan>(), <TextSpan>[]);
// Not even remotely close.
expect(paragraph.hitTest(result = BoxHitTestResult(), position: const Offset(9999.0, 9999.0)), isFalse);
expect(result.path.map((HitTestEntry<HitTestTarget> entry) => entry.target).whereType<TextSpan>(), <TextSpan>[]);
});
test('TextSpan Hit testing with text justification', () {
const TextSpan textSpanA = TextSpan(text: 'A '); // The space is a word break.
const TextSpan textSpanB = TextSpan(text: 'B\u200B'); // The zero-width space is used as a line break.
final TextSpan textSpanC = TextSpan(text: 'C' * 10); // The third span starts a new line since it's too long for the first line.
// The text should look like:
// A B
// CCCCCCCCCC
final TextSpan text = TextSpan(
text: '',
style: const TextStyle(fontSize: 10.0),
children: <InlineSpan>[textSpanA, textSpanB, textSpanC],
);
final RenderParagraph paragraph = RenderParagraph(text, textDirection: TextDirection.ltr, textAlign: TextAlign.justify);
layout(paragraph, constraints: const BoxConstraints.tightFor(width: 100.0));
BoxHitTestResult result;
// Tapping on A.
expect(paragraph.hitTest(result = BoxHitTestResult(), position: const Offset(5.0, 5.0)), isTrue);
expect(result.path.map((HitTestEntry<HitTestTarget> entry) => entry.target).whereType<TextSpan>(), <TextSpan>[textSpanA]);
// Between A and B.
expect(paragraph.hitTest(result = BoxHitTestResult(), position: const Offset(50.0, 5.0)), isTrue);
expect(result.path.map((HitTestEntry<HitTestTarget> entry) => entry.target).whereType<TextSpan>(), <TextSpan>[textSpanA]);
// On B.
expect(paragraph.hitTest(result = BoxHitTestResult(), position: const Offset(95.0, 5.0)), isTrue);
expect(result.path.map((HitTestEntry<HitTestTarget> entry) => entry.target).whereType<TextSpan>(), <TextSpan>[textSpanB]);
});
group('Selection', () {
void selectionParagraph(RenderParagraph paragraph, TextPosition start, TextPosition end) {
for (final Selectable selectable in (paragraph.registrar! as TestSelectionRegistrar).selectables) {
selectable.dispatchSelectionEvent(
SelectionEdgeUpdateEvent.forStart(
globalPosition: paragraph.getOffsetForCaret(start, Rect.zero) + const Offset(0, 5),
),
);
selectable.dispatchSelectionEvent(
SelectionEdgeUpdateEvent.forEnd(
globalPosition: paragraph.getOffsetForCaret(end, Rect.zero) + const Offset(0, 5),
),
);
}
}
test('subscribe to SelectionRegistrar', () {
final TestSelectionRegistrar registrar = TestSelectionRegistrar();
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(text: '1234567'),
textDirection: TextDirection.ltr,
registrar: registrar,
);
expect(registrar.selectables.length, 1);
paragraph.text = const TextSpan(text: '');
expect(registrar.selectables.length, 0);
});
test('paints selection highlight', () async {
final TestSelectionRegistrar registrar = TestSelectionRegistrar();
const Color selectionColor = Color(0xAF6694e8);
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(text: '1234567'),
textDirection: TextDirection.ltr,
registrar: registrar,
selectionColor: selectionColor,
);
layout(paragraph);
final MockPaintingContext paintingContext = MockPaintingContext();
paragraph.paint(paintingContext, Offset.zero);
expect(paintingContext.canvas.drawnRect, isNull);
expect(paintingContext.canvas.drawnRectPaint, isNull);
selectionParagraph(paragraph, const TextPosition(offset: 1), const TextPosition(offset: 5));
paintingContext.canvas.clear();
paragraph.paint(paintingContext, Offset.zero);
expect(paintingContext.canvas.drawnRect, const Rect.fromLTWH(14.0, 0.0, 56.0, 14.0));
expect(paintingContext.canvas.drawnRectPaint!.style, PaintingStyle.fill);
expect(paintingContext.canvas.drawnRectPaint!.color, selectionColor);
// Selection highlight is painted before text.
expect(paintingContext.canvas.drawnItemTypes, <Type>[Rect, ui.Paragraph]);
selectionParagraph(paragraph, const TextPosition(offset: 2), const TextPosition(offset: 4));
paragraph.paint(paintingContext, Offset.zero);
expect(paintingContext.canvas.drawnRect, const Rect.fromLTWH(28.0, 0.0, 28.0, 14.0));
expect(paintingContext.canvas.drawnRectPaint!.style, PaintingStyle.fill);
expect(paintingContext.canvas.drawnRectPaint!.color, selectionColor);
});
// Regression test for https://github.com/flutter/flutter/issues/126652.
test('paints selection when tap at chinese character', () async {
final TestSelectionRegistrar registrar = TestSelectionRegistrar();
const Color selectionColor = Color(0xAF6694e8);
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(text: '你好'),
textDirection: TextDirection.ltr,
registrar: registrar,
selectionColor: selectionColor,
);
layout(paragraph);
final MockPaintingContext paintingContext = MockPaintingContext();
paragraph.paint(paintingContext, Offset.zero);
expect(paintingContext.canvas.drawnRect, isNull);
expect(paintingContext.canvas.drawnRectPaint, isNull);
for (final Selectable selectable in (paragraph.registrar! as TestSelectionRegistrar).selectables) {
selectable.dispatchSelectionEvent(const SelectWordSelectionEvent(globalPosition: Offset(7, 0)));
}
paintingContext.canvas.clear();
paragraph.paint(paintingContext, Offset.zero);
expect(paintingContext.canvas.drawnRect!.isEmpty, false);
expect(paintingContext.canvas.drawnRectPaint!.style, PaintingStyle.fill);
expect(paintingContext.canvas.drawnRectPaint!.color, selectionColor);
}, skip: isBrowser); // https://github.com/flutter/flutter/issues/61016
test('getPositionForOffset works', () async {
final RenderParagraph paragraph = RenderParagraph(const TextSpan(text: '1234567'), textDirection: TextDirection.ltr);
layout(paragraph);
expect(paragraph.getPositionForOffset(const Offset(42.0, 14.0)), const TextPosition(offset: 3));
});
test('can handle select all when contains widget span', () async {
final TestSelectionRegistrar registrar = TestSelectionRegistrar();
final List<RenderBox> renderBoxes = <RenderBox>[
RenderParagraph(const TextSpan(text: 'widget'), textDirection: TextDirection.ltr),
];
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(
children: <InlineSpan>[
TextSpan(text: 'before the span'),
WidgetSpan(child: Text('widget')),
TextSpan(text: 'after the span'),
]
),
textDirection: TextDirection.ltr,
registrar: registrar,
children: renderBoxes,
);
_applyParentData(renderBoxes, paragraph.text);
layout(paragraph);
// The widget span will register to the selection container without going
// through the render paragraph.
expect(registrar.selectables.length, 2);
final Selectable segment1 = registrar.selectables[0];
segment1.dispatchSelectionEvent(const SelectAllSelectionEvent());
final SelectionGeometry geometry1 = segment1.value;
expect(geometry1.hasContent, true);
expect(geometry1.status, SelectionStatus.uncollapsed);
final Selectable segment2 = registrar.selectables[1];
segment2.dispatchSelectionEvent(const SelectAllSelectionEvent());
final SelectionGeometry geometry2 = segment2.value;
expect(geometry2.hasContent, true);
expect(geometry2.status, SelectionStatus.uncollapsed);
});
test('can granularly extend selection - character', () async {
final TestSelectionRegistrar registrar = TestSelectionRegistrar();
final List<RenderBox> renderBoxes = <RenderBox>[];
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(
children: <InlineSpan>[
TextSpan(text: 'how are you\nI am fine\nThank you'),
]
),
textDirection: TextDirection.ltr,
registrar: registrar,
children: renderBoxes,
);
layout(paragraph);
expect(registrar.selectables.length, 1);
selectionParagraph(paragraph, const TextPosition(offset: 4), const TextPosition(offset: 5));
expect(paragraph.selections.length, 1);
TextSelection selection = paragraph.selections[0];
expect(selection.start, 4); // how [a]re you
expect(selection.end, 5);
// Equivalent to sending shift + arrow-right
registrar.selectables[0].dispatchSelectionEvent(
const GranularlyExtendSelectionEvent(
forward: true,
isEnd: true,
granularity: TextGranularity.character,
),
);
selection = paragraph.selections[0];
expect(selection.start, 4); // how [ar]e you
expect(selection.end, 6);
// Equivalent to sending shift + arrow-left
registrar.selectables[0].dispatchSelectionEvent(
const GranularlyExtendSelectionEvent(
forward: false,
isEnd: true,
granularity: TextGranularity.character,
),
);
selection = paragraph.selections[0];
expect(selection.start, 4); // how [a]re you
expect(selection.end, 5);
});
test('can granularly extend selection - word', () async {
final TestSelectionRegistrar registrar = TestSelectionRegistrar();
final List<RenderBox> renderBoxes = <RenderBox>[];
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(
children: <InlineSpan>[
TextSpan(text: 'how are you\nI am fine\nThank you'),
]
),
textDirection: TextDirection.ltr,
registrar: registrar,
children: renderBoxes,
);
layout(paragraph);
expect(registrar.selectables.length, 1);
selectionParagraph(paragraph, const TextPosition(offset: 4), const TextPosition(offset: 5));
expect(paragraph.selections.length, 1);
TextSelection selection = paragraph.selections[0];
expect(selection.start, 4); // how [a]re you
expect(selection.end, 5);
// Equivalent to sending shift + alt + arrow-right.
registrar.selectables[0].dispatchSelectionEvent(
const GranularlyExtendSelectionEvent(
forward: true,
isEnd: true,
granularity: TextGranularity.word,
),
);
selection = paragraph.selections[0];
expect(selection.start, 4); // how [are] you
expect(selection.end, 7);
// Equivalent to sending shift + alt + arrow-left.
registrar.selectables[0].dispatchSelectionEvent(
const GranularlyExtendSelectionEvent(
forward: false,
isEnd: true,
granularity: TextGranularity.word,
),
);
expect(paragraph.selections.length, 1); // how []are you
expect(paragraph.selections[0], const TextSelection.collapsed(offset: 4));
// Equivalent to sending shift + alt + arrow-left.
registrar.selectables[0].dispatchSelectionEvent(
const GranularlyExtendSelectionEvent(
forward: false,
isEnd: true,
granularity: TextGranularity.word,
),
);
selection = paragraph.selections[0];
expect(selection.start, 0); // [how ]are you
expect(selection.end, 4);
});
test('can granularly extend selection - line', () async {
final TestSelectionRegistrar registrar = TestSelectionRegistrar();
final List<RenderBox> renderBoxes = <RenderBox>[];
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(
children: <InlineSpan>[
TextSpan(text: 'how are you\nI am fine\nThank you'),
]
),
textDirection: TextDirection.ltr,
registrar: registrar,
children: renderBoxes,
);
layout(paragraph);
expect(registrar.selectables.length, 1);
selectionParagraph(paragraph, const TextPosition(offset: 4), const TextPosition(offset: 5));
expect(paragraph.selections.length, 1);
TextSelection selection = paragraph.selections[0];
expect(selection.start, 4); // how [a]re you
expect(selection.end, 5);
// Equivalent to sending shift + meta + arrow-right.
registrar.selectables[0].dispatchSelectionEvent(
const GranularlyExtendSelectionEvent(
forward: true,
isEnd: true,
granularity: TextGranularity.line,
),
);
selection = paragraph.selections[0];
// how [are you]
expect(selection, const TextRange(start: 4, end: 11));
// Equivalent to sending shift + meta + arrow-left.
registrar.selectables[0].dispatchSelectionEvent(
const GranularlyExtendSelectionEvent(
forward: false,
isEnd: true,
granularity: TextGranularity.line,
),
);
selection = paragraph.selections[0];
// [how ]are you
expect(selection, const TextRange(start: 0, end: 4));
});
test('can granularly extend selection - document', () async {
final TestSelectionRegistrar registrar = TestSelectionRegistrar();
final List<RenderBox> renderBoxes = <RenderBox>[];
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(
children: <InlineSpan>[
TextSpan(text: 'how are you\nI am fine\nThank you'),
]
),
textDirection: TextDirection.ltr,
registrar: registrar,
children: renderBoxes,
);
layout(paragraph);
expect(registrar.selectables.length, 1);
selectionParagraph(paragraph, const TextPosition(offset: 14), const TextPosition(offset: 15));
expect(paragraph.selections.length, 1);
TextSelection selection = paragraph.selections[0];
// how are you
// I [a]m fine
expect(selection.start, 14);
expect(selection.end, 15);
// Equivalent to sending shift + meta + arrow-down.
registrar.selectables[0].dispatchSelectionEvent(
const GranularlyExtendSelectionEvent(
forward: true,
isEnd: true,
granularity: TextGranularity.document,
),
);
selection = paragraph.selections[0];
// how are you
// I [am fine
// Thank you]
expect(selection.start, 14);
expect(selection.end, 31);
// Equivalent to sending shift + meta + arrow-up.
registrar.selectables[0].dispatchSelectionEvent(
const GranularlyExtendSelectionEvent(
forward: false,
isEnd: true,
granularity: TextGranularity.document,
),
);
selection = paragraph.selections[0];
// [how are you
// I ]am fine
// Thank you
expect(selection.start, 0);
expect(selection.end, 14);
});
test('can granularly extend selection when no active selection', () async {
final TestSelectionRegistrar registrar = TestSelectionRegistrar();
final List<RenderBox> renderBoxes = <RenderBox>[];
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(
children: <InlineSpan>[
TextSpan(text: 'how are you\nI am fine\nThank you'),
]
),
textDirection: TextDirection.ltr,
registrar: registrar,
children: renderBoxes,
);
layout(paragraph);
expect(registrar.selectables.length, 1);
expect(paragraph.selections.length, 0);
// Equivalent to sending shift + alt + right.
registrar.selectables[0].dispatchSelectionEvent(
const GranularlyExtendSelectionEvent(
forward: true,
isEnd: true,
granularity: TextGranularity.word,
),
);
TextSelection selection = paragraph.selections[0];
// [how] are you
// I am fine
// Thank you
expect(selection.start, 0);
expect(selection.end, 3);
// Remove selection
registrar.selectables[0].dispatchSelectionEvent(
const ClearSelectionEvent(),
);
expect(paragraph.selections.length, 0);
// Equivalent to sending shift + alt + left.
registrar.selectables[0].dispatchSelectionEvent(
const GranularlyExtendSelectionEvent(
forward: false,
isEnd: true,
granularity: TextGranularity.word,
),
);
selection = paragraph.selections[0];
// how are you
// I am fine
// Thank [you]
expect(selection.start, 28);
expect(selection.end, 31);
});
test('can directionally extend selection', () async {
final TestSelectionRegistrar registrar = TestSelectionRegistrar();
final List<RenderBox> renderBoxes = <RenderBox>[];
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(
children: <InlineSpan>[
TextSpan(text: 'how are you\nI am fine\nThank you'),
]
),
textDirection: TextDirection.ltr,
registrar: registrar,
children: renderBoxes,
);
layout(paragraph);
expect(registrar.selectables.length, 1);
selectionParagraph(paragraph, const TextPosition(offset: 14), const TextPosition(offset: 15));
expect(paragraph.selections.length, 1);
TextSelection selection = paragraph.selections[0];
// how are you
// I [a]m fine
expect(selection.start, 14);
expect(selection.end, 15);
final Matrix4 transform = registrar.selectables[0].getTransformTo(null);
final double baseline = MatrixUtils.transformPoint(
transform,
registrar.selectables[0].value.endSelectionPoint!.localPosition,
).dx;
// Equivalent to sending shift + arrow-down.
registrar.selectables[0].dispatchSelectionEvent(
DirectionallyExtendSelectionEvent(
isEnd: true,
dx: baseline,
direction: SelectionExtendDirection.nextLine,
),
);
selection = paragraph.selections[0];
// how are you
// I [am fine
// Tha]nk you
expect(selection.start, 14);
expect(selection.end, 25);
// Equivalent to sending shift + arrow-up.
registrar.selectables[0].dispatchSelectionEvent(
DirectionallyExtendSelectionEvent(
isEnd: true,
dx: baseline,
direction: SelectionExtendDirection.previousLine,
),
);
selection = paragraph.selections[0];
// how are you
// I [a]m fine
// Thank you
expect(selection.start, 14);
expect(selection.end, 15);
});
test('can directionally extend selection when no selection', () async {
final TestSelectionRegistrar registrar = TestSelectionRegistrar();
final List<RenderBox> renderBoxes = <RenderBox>[];
final RenderParagraph paragraph = RenderParagraph(
const TextSpan(
children: <InlineSpan>[
TextSpan(text: 'how are you\nI am fine\nThank you'),
]
),
textDirection: TextDirection.ltr,
registrar: registrar,
children: renderBoxes,
);
layout(paragraph);
expect(registrar.selectables.length, 1);
expect(paragraph.selections.length, 0);
final Matrix4 transform = registrar.selectables[0].getTransformTo(null);
final double baseline = MatrixUtils.transformPoint(
transform,
Offset(registrar.selectables[0].size.width / 2, 0),
).dx;
// Equivalent to sending shift + arrow-down.
registrar.selectables[0].dispatchSelectionEvent(
DirectionallyExtendSelectionEvent(
isEnd: true,
dx: baseline,
direction: SelectionExtendDirection.forward,
),
);
TextSelection selection = paragraph.selections[0];
// [how ar]e you
// I am fine
// Thank you
expect(selection.start, 0);
expect(selection.end, 6);
registrar.selectables[0].dispatchSelectionEvent(
const ClearSelectionEvent(),
);
expect(paragraph.selections.length, 0);
// Equivalent to sending shift + arrow-up.
registrar.selectables[0].dispatchSelectionEvent(
DirectionallyExtendSelectionEvent(
isEnd: true,
dx: baseline,
direction: SelectionExtendDirection.backward,
),
);
selection = paragraph.selections[0];
// how are you
// I am fine
// Thank [you]
expect(selection.start, 28);
expect(selection.end, 31);
});
});
test('can just update the gesture recognizer', () async {
final TapGestureRecognizer recognizerBefore = TapGestureRecognizer()..onTap = () {};
final RenderParagraph paragraph = RenderParagraph(
TextSpan(text: 'How are you \n', recognizer: recognizerBefore),
textDirection: TextDirection.ltr,
);
int semanticsUpdateCount = 0;
TestRenderingFlutterBinding.instance.pipelineOwner.ensureSemantics(
listener: () {
++semanticsUpdateCount;
},
);
layout(paragraph);
expect((paragraph.text as TextSpan).recognizer, same(recognizerBefore));
final SemanticsNode nodeBefore = SemanticsNode();
paragraph.assembleSemanticsNode(nodeBefore, SemanticsConfiguration(), <SemanticsNode>[]);
expect(semanticsUpdateCount, 0);
List<SemanticsNode> children = <SemanticsNode>[];
nodeBefore.visitChildren((SemanticsNode child) {
children.add(child);
return true;
});
SemanticsData data = children.single.getSemanticsData();
expect(data.hasAction(SemanticsAction.longPress), false);
expect(data.hasAction(SemanticsAction.tap), true);
final LongPressGestureRecognizer recognizerAfter = LongPressGestureRecognizer()..onLongPress = () {};
paragraph.text = TextSpan(text: 'How are you \n', recognizer: recognizerAfter);
pumpFrame(phase: EnginePhase.flushSemantics);
expect((paragraph.text as TextSpan).recognizer, same(recognizerAfter));
final SemanticsNode nodeAfter = SemanticsNode();
paragraph.assembleSemanticsNode(nodeAfter, SemanticsConfiguration(), <SemanticsNode>[]);
expect(semanticsUpdateCount, 1);
children = <SemanticsNode>[];
nodeAfter.visitChildren((SemanticsNode child) {
children.add(child);
return true;
});
data = children.single.getSemanticsData();
expect(data.hasAction(SemanticsAction.longPress), true);
expect(data.hasAction(SemanticsAction.tap), false);
});
}
class MockCanvas extends Fake implements Canvas {
Rect? drawnRect;
Paint? drawnRectPaint;
List<Type> drawnItemTypes=<Type>[];
@override
void drawRect(Rect rect, Paint paint) {
drawnRect = rect;
drawnRectPaint = paint;
drawnItemTypes.add(Rect);
}
@override
void drawParagraph(ui.Paragraph paragraph, Offset offset) {
drawnItemTypes.add(ui.Paragraph);
}
void clear() {
drawnRect = null;
drawnRectPaint = null;
drawnItemTypes.clear();
}
}
class MockPaintingContext extends Fake implements PaintingContext {
@override
final MockCanvas canvas = MockCanvas();
}
class TestSelectionRegistrar extends SelectionRegistrar {
final List<Selectable> selectables = <Selectable>[];
@override
void add(Selectable selectable) {
selectables.add(selectable);
}
@override
void remove(Selectable selectable) {
expect(selectables.remove(selectable), isTrue);
}
}
| flutter/packages/flutter/test/rendering/paragraph_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/rendering/paragraph_test.dart",
"repo_id": "flutter",
"token_count": 22533
} | 704 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import 'rendering_tester.dart';
void main() {
TestRenderingFlutterBinding.ensureInitialized();
test('Stack can layout with top, right, bottom, left 0.0', () {
final RenderBox box = RenderConstrainedBox(
additionalConstraints: BoxConstraints.tight(const Size(100.0, 100.0)),
);
layout(box, constraints: const BoxConstraints());
expect(box.size.width, equals(100.0));
expect(box.size.height, equals(100.0));
expect(box.size, equals(const Size(100.0, 100.0)));
expect(box.size.runtimeType.toString(), equals('_DebugSize'));
});
}
| flutter/packages/flutter/test/rendering/size_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/rendering/size_test.dart",
"repo_id": "flutter",
"token_count": 282
} | 705 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file is separate from viewport_test.dart because we can't use both
// testWidgets and rendering_tester in the same file - testWidgets will
// initialize a binding, which rendering_tester will attempt to re-initialize
// (or vice versa).
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import 'rendering_tester.dart';
void main() {
TestRenderingFlutterBinding.ensureInitialized();
const double width = 800;
const double height = 600;
Rect rectExpandedOnAxis(double value) => Rect.fromLTRB(0.0, 0.0 - value, width, height + value);
late List<RenderSliver> children;
setUp(() {
children = <RenderSliver>[
RenderSliverToBoxAdapter(
child: RenderSizedBox(const Size(800, 400)),
),
];
});
test('Cache extent - null, pixels', () async {
final RenderViewport renderViewport = RenderViewport(
crossAxisDirection: AxisDirection.left,
offset: ViewportOffset.zero(),
children: children,
);
layout(renderViewport, phase: EnginePhase.flushSemantics);
expect(
renderViewport.describeSemanticsClip(null),
rectExpandedOnAxis(RenderAbstractViewport.defaultCacheExtent),
);
});
test('Cache extent - 0, pixels', () async {
final RenderViewport renderViewport = RenderViewport(
crossAxisDirection: AxisDirection.left,
offset: ViewportOffset.zero(),
cacheExtent: 0.0,
children: children,
);
layout(renderViewport, phase: EnginePhase.flushSemantics);
expect(renderViewport.describeSemanticsClip(null), rectExpandedOnAxis(0.0));
});
test('Cache extent - 500, pixels', () async {
final RenderViewport renderViewport = RenderViewport(
crossAxisDirection: AxisDirection.left,
offset: ViewportOffset.zero(),
cacheExtent: 500.0,
children: children,
);
layout(renderViewport, phase: EnginePhase.flushSemantics);
expect(renderViewport.describeSemanticsClip(null), rectExpandedOnAxis(500.0));
});
test('Cache extent - nullx viewport', () async {
await expectLater(
() => RenderViewport(
crossAxisDirection: AxisDirection.left,
offset: ViewportOffset.zero(),
cacheExtentStyle: CacheExtentStyle.viewport,
children: children,
),
throwsAssertionError,
);
});
test('Cache extent - 0x viewport', () async {
final RenderViewport renderViewport = RenderViewport(
crossAxisDirection: AxisDirection.left,
offset: ViewportOffset.zero(),
cacheExtent: 0.0,
cacheExtentStyle: CacheExtentStyle.viewport,
children: children,
);
layout(renderViewport);
expect(renderViewport.describeSemanticsClip(null), rectExpandedOnAxis(0));
});
test('Cache extent - .5x viewport', () async {
final RenderViewport renderViewport = RenderViewport(
crossAxisDirection: AxisDirection.left,
offset: ViewportOffset.zero(),
cacheExtent: .5,
cacheExtentStyle: CacheExtentStyle.viewport,
children: children,
);
layout(renderViewport);
expect(renderViewport.describeSemanticsClip(null), rectExpandedOnAxis(height / 2));
});
test('Cache extent - 1x viewport', () async {
final RenderViewport renderViewport = RenderViewport(
crossAxisDirection: AxisDirection.left,
offset: ViewportOffset.zero(),
cacheExtent: 1.0,
cacheExtentStyle: CacheExtentStyle.viewport,
children: children,
);
layout(renderViewport);
expect(renderViewport.describeSemanticsClip(null), rectExpandedOnAxis(height));
});
test('Cache extent - 2.5x viewport', () async {
final RenderViewport renderViewport = RenderViewport(
crossAxisDirection: AxisDirection.left,
offset: ViewportOffset.zero(),
cacheExtent: 2.5,
cacheExtentStyle: CacheExtentStyle.viewport,
children: children,
);
layout(renderViewport);
expect(renderViewport.describeSemanticsClip(null), rectExpandedOnAxis(height * 2.5));
});
test('RenderShrinkWrappingViewport describeApproximatePaintClip with infinite viewportMainAxisExtent returns finite rect', () {
final RenderSliver child = CustomConstraintsRenderSliver(const SliverConstraints(
axisDirection: AxisDirection.down,
cacheOrigin: 0.0,
crossAxisDirection: AxisDirection.left,
crossAxisExtent: 400.0,
growthDirection: GrowthDirection.forward,
overlap: 1.0, // must not equal 0 for this test
precedingScrollExtent: 0.0,
remainingPaintExtent: double.infinity,
remainingCacheExtent: 0.0,
scrollOffset: 0.0,
userScrollDirection: ScrollDirection.idle,
viewportMainAxisExtent: double.infinity, // must == infinity
));
final RenderShrinkWrappingViewport viewport = RenderShrinkWrappingViewport(
crossAxisDirection: AxisDirection.left,
offset: ViewportOffset.zero(),
children: <RenderSliver>[ child ],
);
layout(viewport);
expect(
viewport.describeApproximatePaintClip(child),
const Rect.fromLTRB(0.0, 0.0, 800.0, 600.0),
);
});
}
class CustomConstraintsRenderSliver extends RenderSliver {
CustomConstraintsRenderSliver(this.constraints);
@override
SliverGeometry get geometry => SliverGeometry.zero;
@override
final SliverConstraints constraints;
@override
void performLayout() {}
}
| flutter/packages/flutter/test/rendering/viewport_caching_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/rendering/viewport_caching_test.dart",
"repo_id": "flutter",
"token_count": 2028
} | 706 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/semantics.dart';
import 'package:flutter_test/flutter_test.dart';
import '../widgets/semantics_tester.dart';
void main() {
testWidgets('Performing SemanticsAction.showOnScreen does not crash if node no longer exist', (WidgetTester tester) async {
// Regression test for https://github.com/flutter/flutter/issues/100358.
final SemanticsTester semantics = SemanticsTester(tester);
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Semantics(
explicitChildNodes: true,
child: const Text('Hello World'),
),
),
);
final int nodeId = tester.semantics.find(find.bySemanticsLabel('Hello World')).id;
await tester.pumpWidget(
Directionality(
textDirection: TextDirection.ltr,
child: Container(),
),
);
// Node with $nodeId is no longer in the tree.
expect(semantics, isNot(hasSemantics(TestSemantics(id: nodeId))));
// Executing SemanticsAction.showOnScreen on that node does not crash.
// (A platform may not have processed the semantics update yet and send
// actions for no longer existing nodes.)
tester.binding.performSemanticsAction(SemanticsActionEvent(
type: SemanticsAction.showOnScreen,
nodeId: nodeId,
viewId: tester.view.viewId,
));
semantics.dispose();
});
}
| flutter/packages/flutter/test/semantics/semantics_owner_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/semantics/semantics_owner_test.dart",
"repo_id": "flutter",
"token_count": 570
} | 707 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/foundation.dart';
import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
/// Used in internal testing.
class FakePlatformViewController extends PlatformViewController {
FakePlatformViewController(this.viewId);
bool disposed = false;
bool focusCleared = false;
/// Events that are dispatched.
List<PointerEvent> dispatchedPointerEvents = <PointerEvent>[];
@override
final int viewId;
@override
Future<void> dispatchPointerEvent(PointerEvent event) async {
dispatchedPointerEvents.add(event);
}
void clearTestingVariables() {
dispatchedPointerEvents.clear();
disposed = false;
focusCleared = false;
}
@override
Future<void> dispose() async {
disposed = true;
}
@override
Future<void> clearFocus() async {
focusCleared = true;
}
}
class FakeAndroidViewController implements AndroidViewController {
FakeAndroidViewController(
this.viewId, {
this.requiresSize = false,
this.requiresViewComposition = false,
});
bool disposed = false;
bool focusCleared = false;
bool created = false;
// If true, [create] won't be considered to have been called successfully
// unless it includes a size.
bool requiresSize;
bool _createCalledSuccessfully = false;
Offset? createPosition;
final List<PlatformViewCreatedCallback> _createdCallbacks = <PlatformViewCreatedCallback>[];
/// Events that are dispatched.
List<PointerEvent> dispatchedPointerEvents = <PointerEvent>[];
@override
final int viewId;
@override
late PointTransformer pointTransformer;
@override
Future<void> dispatchPointerEvent(PointerEvent event) async {
dispatchedPointerEvents.add(event);
}
void clearTestingVariables() {
dispatchedPointerEvents.clear();
disposed = false;
focusCleared = false;
}
@override
Future<void> dispose() async {
disposed = true;
}
@override
Future<void> clearFocus() async {
focusCleared = true;
}
@override
Future<Size> setSize(Size size) {
return Future<Size>.value(size);
}
@override
Future<void> setOffset(Offset off) async {}
@override
int get textureId => 0;
@override
bool get awaitingCreation => !_createCalledSuccessfully;
@override
bool get isCreated => created;
@override
void addOnPlatformViewCreatedListener(PlatformViewCreatedCallback listener) {
created = true;
createdCallbacks.add(listener);
}
@override
void removeOnPlatformViewCreatedListener(PlatformViewCreatedCallback listener) {
createdCallbacks.remove(listener);
}
@override
Future<void> sendMotionEvent(AndroidMotionEvent event) {
throw UnimplementedError();
}
@override
Future<void> setLayoutDirection(TextDirection layoutDirection) {
throw UnimplementedError();
}
@override
Future<void> create({Size? size, Offset? position}) async {
assert(!_createCalledSuccessfully);
if (requiresSize && size != null) {
assert(!size.isEmpty);
}
_createCalledSuccessfully = size != null && position != null || !requiresSize;
createPosition = position;
}
@override
List<PlatformViewCreatedCallback> get createdCallbacks => _createdCallbacks;
@override
bool requiresViewComposition;
}
class FakeAndroidPlatformViewsController {
FakeAndroidPlatformViewsController() {
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger.setMockMethodCallHandler(SystemChannels.platform_views, _onMethodCall);
}
Iterable<FakeAndroidPlatformView> get views => _views.values;
final Map<int, FakeAndroidPlatformView> _views = <int, FakeAndroidPlatformView>{};
final Map<int, List<FakeAndroidMotionEvent>> motionEvents = <int, List<FakeAndroidMotionEvent>>{};
final Set<String> _registeredViewTypes = <String>{};
int _textureCounter = 0;
Completer<void>? resizeCompleter;
Completer<void>? createCompleter;
int? lastClearedFocusViewId;
Map<int, Offset> offsets = <int, Offset>{};
/// True if Texture Layer Hybrid Composition mode should be enabled.
///
/// When false, `create` will simulate the engine's fallback mode.
bool allowTextureLayerMode = true;
void registerViewType(String viewType) {
_registeredViewTypes.add(viewType);
}
void invokeViewFocused(int viewId) {
final MethodCodec codec = SystemChannels.platform_views.codec;
final ByteData data = codec.encodeMethodCall(MethodCall('viewFocused', viewId));
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger
.handlePlatformMessage(SystemChannels.platform_views.name, data, (ByteData? data) {});
}
Future<dynamic> _onMethodCall(MethodCall call) {
return switch (call.method) {
'create' => _create(call),
'dispose' => _dispose(call),
'resize' => _resize(call),
'touch' => _touch(call),
'setDirection' => _setDirection(call),
'clearFocus' => _clearFocus(call),
'offset' => _offset(call),
_ => Future<dynamic>.sync(() => null),
};
}
Future<dynamic> _create(MethodCall call) async {
final Map<dynamic, dynamic> args = call.arguments as Map<dynamic, dynamic>;
final int id = args['id'] as int;
final String viewType = args['viewType'] as String;
final double? width = args['width'] as double?;
final double? height = args['height'] as double?;
final int layoutDirection = args['direction'] as int;
final bool? hybrid = args['hybrid'] as bool?;
final bool? hybridFallback = args['hybridFallback'] as bool?;
final Uint8List? creationParams = args['params'] as Uint8List?;
final double? top = args['top'] as double?;
final double? left = args['left'] as double?;
if (_views.containsKey(id)) {
throw PlatformException(
code: 'error',
message: 'Trying to create an already created platform view, view id: $id',
);
}
if (!_registeredViewTypes.contains(viewType)) {
throw PlatformException(
code: 'error',
message: 'Trying to create a platform view of unregistered type: $viewType',
);
}
if (createCompleter != null) {
await createCompleter!.future;
}
_views[id] = FakeAndroidPlatformView(id, viewType,
width != null && height != null ? Size(width, height) : null,
layoutDirection,
hybrid: hybrid,
hybridFallback: hybridFallback,
creationParams: creationParams,
position: left != null && top != null ? Offset(left, top) : null,
);
// Return a hybrid result (null rather than a texture ID) if:
final bool hybridResult =
// hybrid was explicitly requested, or
(hybrid ?? false) ||
// hybrid fallback was requested and simulated.
(!allowTextureLayerMode && (hybridFallback ?? false));
if (hybridResult) {
return Future<void>.value();
}
final int textureId = _textureCounter++;
return Future<int>.value(textureId);
}
Future<dynamic> _dispose(MethodCall call) {
assert(call.arguments is Map);
final Map<Object?, Object?> arguments = call.arguments as Map<Object?, Object?>;
final int id = arguments['id']! as int;
final bool hybrid = arguments['hybrid']! as bool;
if (hybrid && !_views[id]!.hybrid!) {
throw ArgumentError('An $AndroidViewController using hybrid composition must pass `hybrid: true`');
} else if (!hybrid && (_views[id]!.hybrid ?? false)) {
throw ArgumentError('An $AndroidViewController not using hybrid composition must pass `hybrid: false`');
}
if (!_views.containsKey(id)) {
throw PlatformException(
code: 'error',
message: 'Trying to dispose a platform view with unknown id: $id',
);
}
_views.remove(id);
return Future<dynamic>.sync(() => null);
}
Future<dynamic> _resize(MethodCall call) async {
final Map<dynamic, dynamic> args = call.arguments as Map<dynamic, dynamic>;
final int id = args['id'] as int;
final double width = args['width'] as double;
final double height = args['height'] as double;
if (!_views.containsKey(id)) {
throw PlatformException(
code: 'error',
message: 'Trying to resize a platform view with unknown id: $id',
);
}
if (resizeCompleter != null) {
await resizeCompleter!.future;
}
_views[id] = _views[id]!.copyWith(size: Size(width, height));
return Future<Map<dynamic, dynamic>>.sync(() => <dynamic, dynamic>{'width': width, 'height': height});
}
Future<dynamic> _offset(MethodCall call) async {
final Map<dynamic, dynamic> args = call.arguments as Map<dynamic, dynamic>;
final int id = args['id'] as int;
final double top = args['top'] as double;
final double left = args['left'] as double;
offsets[id] = Offset(left, top);
return Future<dynamic>.sync(() => null);
}
Future<dynamic> _touch(MethodCall call) {
final List<dynamic> args = call.arguments as List<dynamic>;
final int id = args[0] as int;
final int action = args[3] as int;
final List<List<dynamic>> pointerProperties = (args[5] as List<dynamic>).cast<List<dynamic>>();
final List<List<dynamic>> pointerCoords = (args[6] as List<dynamic>).cast<List<dynamic>>();
final List<Offset> pointerOffsets = <Offset> [];
final List<int> pointerIds = <int> [];
for (int i = 0; i < pointerCoords.length; i++) {
pointerIds.add(pointerProperties[i][0] as int);
final double x = pointerCoords[i][7] as double;
final double y = pointerCoords[i][8] as double;
pointerOffsets.add(Offset(x, y));
}
if (!motionEvents.containsKey(id)) {
motionEvents[id] = <FakeAndroidMotionEvent> [];
}
motionEvents[id]!.add(FakeAndroidMotionEvent(action, pointerIds, pointerOffsets));
return Future<dynamic>.sync(() => null);
}
Future<dynamic> _setDirection(MethodCall call) async {
final Map<dynamic, dynamic> args = call.arguments as Map<dynamic, dynamic>;
final int id = args['id'] as int;
final int layoutDirection = args['direction'] as int;
if (!_views.containsKey(id)) {
throw PlatformException(
code: 'error',
message: 'Trying to resize a platform view with unknown id: $id',
);
}
_views[id] = _views[id]!.copyWith(layoutDirection: layoutDirection);
return Future<dynamic>.sync(() => null);
}
Future<dynamic> _clearFocus(MethodCall call) {
final int id = call.arguments as int;
if (!_views.containsKey(id)) {
throw PlatformException(
code: 'error',
message: 'Trying to clear the focus on a platform view with unknown id: $id',
);
}
lastClearedFocusViewId = id;
return Future<dynamic>.sync(() => null);
}
}
class FakeIosPlatformViewsController {
FakeIosPlatformViewsController() {
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger.setMockMethodCallHandler(SystemChannels.platform_views, _onMethodCall);
}
Iterable<FakeUiKitView> get views => _views.values;
final Map<int, FakeUiKitView> _views = <int, FakeUiKitView>{};
final Set<String> _registeredViewTypes = <String>{};
// When this completer is non null, the 'create' method channel call will be
// delayed until it completes.
Completer<void>? creationDelay;
// Maps a view id to the number of gestures it accepted so far.
final Map<int, int> gesturesAccepted = <int, int>{};
// Maps a view id to the number of gestures it rejected so far.
final Map<int, int> gesturesRejected = <int, int>{};
void registerViewType(String viewType) {
_registeredViewTypes.add(viewType);
}
void invokeViewFocused(int viewId) {
final MethodCodec codec = SystemChannels.platform_views.codec;
final ByteData data = codec.encodeMethodCall(MethodCall('viewFocused', viewId));
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger
.handlePlatformMessage(SystemChannels.platform_views.name, data, (ByteData? data) {});
}
Future<dynamic> _onMethodCall(MethodCall call) {
return switch (call.method) {
'create' => _create(call),
'dispose' => _dispose(call),
'acceptGesture' => _acceptGesture(call),
'rejectGesture' => _rejectGesture(call),
_ => Future<dynamic>.sync(() => null),
};
}
Future<dynamic> _create(MethodCall call) async {
if (creationDelay != null) {
await creationDelay!.future;
}
final Map<dynamic, dynamic> args = call.arguments as Map<dynamic, dynamic>;
final int id = args['id'] as int;
final String viewType = args['viewType'] as String;
final Uint8List? creationParams = args['params'] as Uint8List?;
if (_views.containsKey(id)) {
throw PlatformException(
code: 'error',
message: 'Trying to create an already created platform view, view id: $id',
);
}
if (!_registeredViewTypes.contains(viewType)) {
throw PlatformException(
code: 'error',
message: 'Trying to create a platform view of unregistered type: $viewType',
);
}
_views[id] = FakeUiKitView(id, viewType, creationParams);
gesturesAccepted[id] = 0;
gesturesRejected[id] = 0;
return Future<int?>.sync(() => null);
}
Future<dynamic> _acceptGesture(MethodCall call) async {
final Map<dynamic, dynamic> args = call.arguments as Map<dynamic, dynamic>;
final int id = args['id'] as int;
gesturesAccepted[id] = gesturesAccepted[id]! + 1;
return Future<int?>.sync(() => null);
}
Future<dynamic> _rejectGesture(MethodCall call) async {
final Map<dynamic, dynamic> args = call.arguments as Map<dynamic, dynamic>;
final int id = args['id'] as int;
gesturesRejected[id] = gesturesRejected[id]! + 1;
return Future<int?>.sync(() => null);
}
Future<dynamic> _dispose(MethodCall call) {
final int id = call.arguments as int;
if (!_views.containsKey(id)) {
throw PlatformException(
code: 'error',
message: 'Trying to dispose a platform view with unknown id: $id',
);
}
_views.remove(id);
return Future<dynamic>.sync(() => null);
}
}
class FakeMacosPlatformViewsController {
FakeMacosPlatformViewsController() {
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger.setMockMethodCallHandler(SystemChannels.platform_views, _onMethodCall);
}
Iterable<FakeAppKitView> get views => _views.values;
final Map<int, FakeAppKitView> _views = <int, FakeAppKitView>{};
final Set<String> _registeredViewTypes = <String>{};
// When this completer is non null, the 'create' method channel call will be
// delayed until it completes.
Completer<void>? creationDelay;
// Maps a view id to the number of gestures it accepted so far.
final Map<int, int> gesturesAccepted = <int, int>{};
// Maps a view id to the number of gestures it rejected so far.
final Map<int, int> gesturesRejected = <int, int>{};
void registerViewType(String viewType) {
_registeredViewTypes.add(viewType);
}
void invokeViewFocused(int viewId) {
final MethodCodec codec = SystemChannels.platform_views.codec;
final ByteData data = codec.encodeMethodCall(MethodCall('viewFocused', viewId));
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger
.handlePlatformMessage(SystemChannels.platform_views.name, data, (ByteData? data) {});
}
Future<dynamic> _onMethodCall(MethodCall call) {
return switch (call.method) {
'create' => _create(call),
'dispose' => _dispose(call),
'acceptGesture' => _acceptGesture(call),
'rejectGesture' => _rejectGesture(call),
_ => Future<dynamic>.sync(() => null),
};
}
Future<dynamic> _create(MethodCall call) async {
if (creationDelay != null) {
await creationDelay!.future;
}
final Map<dynamic, dynamic> args = call.arguments as Map<dynamic, dynamic>;
final int id = args['id'] as int;
final String viewType = args['viewType'] as String;
final Uint8List? creationParams = args['params'] as Uint8List?;
if (_views.containsKey(id)) {
throw PlatformException(
code: 'error',
message: 'Trying to create an already created platform view, view id: $id',
);
}
if (!_registeredViewTypes.contains(viewType)) {
throw PlatformException(
code: 'error',
message: 'Trying to create a platform view of unregistered type: $viewType',
);
}
_views[id] = FakeAppKitView(id, viewType, creationParams);
gesturesAccepted[id] = 0;
gesturesRejected[id] = 0;
return Future<int?>.sync(() => null);
}
Future<dynamic> _acceptGesture(MethodCall call) async {
final Map<dynamic, dynamic> args = call.arguments as Map<dynamic, dynamic>;
final int id = args['id'] as int;
gesturesAccepted[id] = gesturesAccepted[id]! + 1;
return Future<int?>.sync(() => null);
}
Future<dynamic> _rejectGesture(MethodCall call) async {
final Map<dynamic, dynamic> args = call.arguments as Map<dynamic, dynamic>;
final int id = args['id'] as int;
gesturesRejected[id] = gesturesRejected[id]! + 1;
return Future<int?>.sync(() => null);
}
Future<dynamic> _dispose(MethodCall call) {
final int id = call.arguments as int;
if (!_views.containsKey(id)) {
throw PlatformException(
code: 'error',
message: 'Trying to dispose a platform view with unknown id: $id',
);
}
_views.remove(id);
return Future<dynamic>.sync(() => null);
}
}
@immutable
class FakeAndroidPlatformView {
const FakeAndroidPlatformView(this.id, this.type, this.size, this.layoutDirection,
{this.hybrid, this.hybridFallback, this.creationParams, this.position});
final int id;
final String type;
final Uint8List? creationParams;
final Size? size;
final int layoutDirection;
final bool? hybrid;
final bool? hybridFallback;
final Offset? position;
FakeAndroidPlatformView copyWith({Size? size, int? layoutDirection}) => FakeAndroidPlatformView(
id,
type,
size ?? this.size,
layoutDirection ?? this.layoutDirection,
hybrid: hybrid,
hybridFallback: hybridFallback,
creationParams: creationParams,
position: position,
);
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is FakeAndroidPlatformView
&& other.id == id
&& other.type == type
&& listEquals<int>(other.creationParams, creationParams)
&& other.size == size
&& other.hybrid == hybrid
&& other.hybridFallback == hybridFallback
&& other.layoutDirection == layoutDirection
&& other.position == position;
}
@override
int get hashCode => Object.hash(
id,
type,
creationParams == null ? null : Object.hashAll(creationParams!),
size,
layoutDirection,
hybrid,
hybridFallback,
position,
);
@override
String toString() {
return 'FakeAndroidPlatformView(id: $id, type: $type, size: $size, '
'layoutDirection: $layoutDirection, hybrid: $hybrid, '
'hybridFallback: $hybridFallback, creationParams: $creationParams, position: $position)';
}
}
@immutable
class FakeAndroidMotionEvent {
const FakeAndroidMotionEvent(this.action, this.pointerIds, this.pointers);
final int action;
final List<Offset> pointers;
final List<int> pointerIds;
@override
bool operator ==(Object other) {
return other is FakeAndroidMotionEvent
&& listEquals<int>(other.pointerIds, pointerIds)
&& other.action == action
&& listEquals<Offset>(other.pointers, pointers);
}
@override
int get hashCode => Object.hash(action, Object.hashAll(pointers), Object.hashAll(pointerIds));
@override
String toString() {
return 'FakeAndroidMotionEvent(action: $action, pointerIds: $pointerIds, pointers: $pointers)';
}
}
@immutable
class FakeUiKitView {
const FakeUiKitView(this.id, this.type, [this.creationParams]);
final int id;
final String type;
final Uint8List? creationParams;
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is FakeUiKitView
&& other.id == id
&& other.type == type
&& other.creationParams == creationParams;
}
@override
int get hashCode => Object.hash(id, type);
@override
String toString() {
return 'FakeUiKitView(id: $id, type: $type, creationParams: $creationParams)';
}
}
@immutable
class FakeAppKitView {
const FakeAppKitView(this.id, this.type, [this.creationParams]);
final int id;
final String type;
final Uint8List? creationParams;
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is FakeAppKitView
&& other.id == id
&& other.type == type
&& other.creationParams == creationParams;
}
@override
int get hashCode => Object.hash(id, type);
@override
String toString() {
return 'FakeAppKitView(id: $id, type: $type, creationParams: $creationParams)';
}
}
| flutter/packages/flutter/test/services/fake_platform_views.dart/0 | {
"file_path": "flutter/packages/flutter/test/services/fake_platform_views.dart",
"repo_id": "flutter",
"token_count": 7564
} | 708 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
class MockRestorationManager extends TestRestorationManager {
MockRestorationManager({ this.enableChannels = false });
bool get updateScheduled => _updateScheduled;
bool _updateScheduled = false;
final List<RestorationBucket> _buckets = <RestorationBucket>[];
final bool enableChannels;
@override
void initChannels() {
if (enableChannels) {
super.initChannels();
}
}
@override
void scheduleSerializationFor(RestorationBucket bucket) {
_updateScheduled = true;
_buckets.add(bucket);
}
@override
bool unscheduleSerializationFor(RestorationBucket bucket) {
_updateScheduled = true;
return _buckets.remove(bucket);
}
void doSerialization() {
_updateScheduled = false;
for (final RestorationBucket bucket in _buckets) {
bucket.finalize();
}
_buckets.clear();
}
@override
void restoreFrom(TestRestorationData data) {
// Ignore in mock.
}
int rootBucketAccessed = 0;
@override
Future<RestorationBucket?> get rootBucket {
rootBucketAccessed++;
return _rootBucket;
}
late Future<RestorationBucket?> _rootBucket;
set rootBucket(Future<RestorationBucket?> value) {
_rootBucket = value;
_isRestoring = true;
ServicesBinding.instance.addPostFrameCallback((Duration _) {
_isRestoring = false;
});
notifyListeners();
}
@override
bool get isReplacing => _isRestoring;
bool _isRestoring = false;
@override
Future<void> sendToEngine(Uint8List encodedData) {
throw UnimplementedError('unimplemented in mock');
}
@override
String toString() => 'MockManager';
}
const String childrenMapKey = 'c';
const String valuesMapKey = 'v';
| flutter/packages/flutter/test/services/restoration.dart/0 | {
"file_path": "flutter/packages/flutter/test/services/restoration.dart",
"repo_id": "flutter",
"token_count": 665
} | 709 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Align smoke test', (WidgetTester tester) async {
await tester.pumpWidget(
Align(
alignment: const Alignment(0.50, 0.50),
child: Container(),
),
);
await tester.pumpWidget(
Align(
child: Container(),
),
);
await tester.pumpWidget(
const Align(
alignment: Alignment.topLeft,
),
);
await tester.pumpWidget(const Directionality(
textDirection: TextDirection.rtl,
child: Align(
alignment: AlignmentDirectional.topStart,
),
));
await tester.pumpWidget(
const Align(
alignment: Alignment.topLeft,
),
);
});
testWidgets('Align control test (LTR)', (WidgetTester tester) async {
await tester.pumpWidget(const Directionality(
textDirection: TextDirection.ltr,
child: Align(
alignment: AlignmentDirectional.topStart,
child: SizedBox(width: 100.0, height: 80.0),
),
));
expect(tester.getTopLeft(find.byType(SizedBox)).dx, 0.0);
expect(tester.getBottomRight(find.byType(SizedBox)).dx, 100.0);
await tester.pumpWidget(const Directionality(
textDirection: TextDirection.ltr,
child: Align(
alignment: Alignment.topLeft,
child: SizedBox(width: 100.0, height: 80.0),
),
));
expect(tester.getTopLeft(find.byType(SizedBox)).dx, 0.0);
expect(tester.getBottomRight(find.byType(SizedBox)).dx, 100.0);
});
testWidgets('Align control test (RTL)', (WidgetTester tester) async {
await tester.pumpWidget(const Directionality(
textDirection: TextDirection.rtl,
child: Align(
alignment: AlignmentDirectional.topStart,
child: SizedBox(width: 100.0, height: 80.0),
),
));
expect(tester.getTopLeft(find.byType(SizedBox)).dx, 700.0);
expect(tester.getBottomRight(find.byType(SizedBox)).dx, 800.0);
await tester.pumpWidget(const Directionality(
textDirection: TextDirection.ltr,
child: Align(
alignment: Alignment.topLeft,
child: SizedBox(width: 100.0, height: 80.0),
),
));
expect(tester.getTopLeft(find.byType(SizedBox)).dx, 0.0);
expect(tester.getBottomRight(find.byType(SizedBox)).dx, 100.0);
});
testWidgets('Shrink wraps in finite space', (WidgetTester tester) async {
final GlobalKey alignKey = GlobalKey();
await tester.pumpWidget(
SingleChildScrollView(
child: Align(
key: alignKey,
child: const SizedBox(
width: 10.0,
height: 10.0,
),
),
),
);
final Size size = alignKey.currentContext!.size!;
expect(size.width, equals(800.0));
expect(size.height, equals(10.0));
});
testWidgets('Align widthFactor', (WidgetTester tester) async {
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: Row(
crossAxisAlignment: CrossAxisAlignment.start,
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Align(
widthFactor: 0.5,
child: SizedBox(
height: 100.0,
width: 100.0,
),
),
],
),
),
);
final RenderBox box = tester.renderObject<RenderBox>(find.byType(Align));
expect(box.size.width, equals(50.0));
});
testWidgets('Align heightFactor', (WidgetTester tester) async {
await tester.pumpWidget(
const Directionality(
textDirection: TextDirection.ltr,
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Align(
heightFactor: 0.5,
child: SizedBox(
height: 100.0,
width: 100.0,
),
),
],
),
),
);
final RenderBox box = tester.renderObject<RenderBox>(find.byType(Align));
expect(box.size.height, equals(50.0));
});
}
| flutter/packages/flutter/test/widgets/align_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/align_test.dart",
"repo_id": "flutter",
"token_count": 1984
} | 710 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
class TestIntent extends Intent {
const TestIntent();
}
class TestAction extends Action<Intent> {
TestAction();
int calls = 0;
@override
void invoke(Intent intent) {
calls += 1;
}
}
void main() {
testWidgets('WidgetsApp with builder only', (WidgetTester tester) async {
final GlobalKey key = GlobalKey();
await tester.pumpWidget(
WidgetsApp(
key: key,
builder: (BuildContext context, Widget? child) {
return const Placeholder();
},
color: const Color(0xFF123456),
),
);
expect(find.byKey(key), findsOneWidget);
});
testWidgets('WidgetsApp default key bindings', (WidgetTester tester) async {
bool? checked = false;
final GlobalKey key = GlobalKey();
await tester.pumpWidget(
WidgetsApp(
key: key,
builder: (BuildContext context, Widget? child) {
return Material(
child: Checkbox(
value: checked,
autofocus: true,
onChanged: (bool? value) {
checked = value;
},
),
);
},
color: const Color(0xFF123456),
),
);
await tester.pump(); // Wait for focus to take effect.
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Default key mapping worked.
expect(checked, isTrue);
});
testWidgets('WidgetsApp can override default key bindings', (WidgetTester tester) async {
final TestAction action = TestAction();
bool? checked = false;
final GlobalKey key = GlobalKey();
await tester.pumpWidget(
WidgetsApp(
key: key,
actions: <Type, Action<Intent>>{
TestIntent: action,
},
shortcuts: const <ShortcutActivator, Intent> {
SingleActivator(LogicalKeyboardKey.space): TestIntent(),
},
builder: (BuildContext context, Widget? child) {
return Material(
child: Checkbox(
value: checked,
autofocus: true,
onChanged: (bool? value) {
checked = value;
},
),
);
},
color: const Color(0xFF123456),
),
);
await tester.pump();
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
// Default key mapping was not invoked.
expect(checked, isFalse);
// Overridden mapping was invoked.
expect(action.calls, equals(1));
});
testWidgets('WidgetsApp default activation key mappings work', (WidgetTester tester) async {
bool? checked = false;
await tester.pumpWidget(
WidgetsApp(
builder: (BuildContext context, Widget? child) {
return Material(
child: Checkbox(
value: checked,
autofocus: true,
onChanged: (bool? value) {
checked = value;
},
),
);
},
color: const Color(0xFF123456),
),
);
await tester.pump();
// Test three default buttons for the activation action.
await tester.sendKeyEvent(LogicalKeyboardKey.space);
await tester.pumpAndSettle();
expect(checked, isTrue);
// Only space is used as an activation key on web.
if (kIsWeb) {
return;
}
checked = false;
await tester.sendKeyEvent(LogicalKeyboardKey.enter);
await tester.pumpAndSettle();
expect(checked, isTrue);
checked = false;
await tester.sendKeyEvent(LogicalKeyboardKey.numpadEnter);
await tester.pumpAndSettle();
expect(checked, isTrue);
checked = false;
await tester.sendKeyEvent(LogicalKeyboardKey.gameButtonA);
await tester.pumpAndSettle();
expect(checked, isTrue);
checked = false;
await tester.sendKeyEvent(LogicalKeyboardKey.select);
await tester.pumpAndSettle();
expect(checked, isTrue);
}, variant: KeySimulatorTransitModeVariant.all());
group('error control test', () {
Future<void> expectFlutterError({
required GlobalKey<NavigatorState> key,
required Widget widget,
required WidgetTester tester,
required String errorMessage,
}) async {
await tester.pumpWidget(widget);
late FlutterError error;
try {
key.currentState!.pushNamed('/path');
} on FlutterError catch (e) {
error = e;
} finally {
expect(error, isNotNull);
expect(error, isFlutterError);
expect(error.toStringDeep(), errorMessage);
}
}
testWidgets('push unknown route when onUnknownRoute is null', (WidgetTester tester) async {
final GlobalKey<NavigatorState> key = GlobalKey<NavigatorState>();
expectFlutterError(
key: key,
tester: tester,
widget: MaterialApp(
navigatorKey: key,
home: Container(),
onGenerateRoute: (_) => null,
),
errorMessage:
'FlutterError\n'
' Could not find a generator for route RouteSettings("/path", null)\n'
' in the _WidgetsAppState.\n'
' Make sure your root app widget has provided a way to generate\n'
' this route.\n'
' Generators for routes are searched for in the following order:\n'
' 1. For the "/" route, the "home" property, if non-null, is used.\n'
' 2. Otherwise, the "routes" table is used, if it has an entry for\n'
' the route.\n'
' 3. Otherwise, onGenerateRoute is called. It should return a\n'
' non-null value for any valid route not handled by "home" and\n'
' "routes".\n'
' 4. Finally if all else fails onUnknownRoute is called.\n'
' Unfortunately, onUnknownRoute was not set.\n',
);
});
testWidgets('push unknown route when onUnknownRoute returns null', (WidgetTester tester) async {
final GlobalKey<NavigatorState> key = GlobalKey<NavigatorState>();
expectFlutterError(
key: key,
tester: tester,
widget: MaterialApp(
navigatorKey: key,
home: Container(),
onGenerateRoute: (_) => null,
onUnknownRoute: (_) => null,
),
errorMessage:
'FlutterError\n'
' The onUnknownRoute callback returned null.\n'
' When the _WidgetsAppState requested the route\n'
' RouteSettings("/path", null) from its onUnknownRoute callback,\n'
' the callback returned null. Such callbacks must never return\n'
' null.\n' ,
);
});
});
testWidgets('WidgetsApp can customize initial routes', (WidgetTester tester) async {
final GlobalKey<NavigatorState> navigatorKey = GlobalKey<NavigatorState>();
await tester.pumpWidget(
WidgetsApp(
navigatorKey: navigatorKey,
onGenerateInitialRoutes: (String initialRoute) {
expect(initialRoute, '/abc');
return <Route<void>>[
PageRouteBuilder<void>(
pageBuilder: (
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
) {
return const Text('non-regular page one');
},
),
PageRouteBuilder<void>(
pageBuilder: (
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
) {
return const Text('non-regular page two');
},
),
];
},
initialRoute: '/abc',
onGenerateRoute: (RouteSettings settings) {
return PageRouteBuilder<void>(
pageBuilder: (
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
) {
return const Text('regular page');
},
);
},
color: const Color(0xFF123456),
),
);
expect(find.text('non-regular page two'), findsOneWidget);
expect(find.text('non-regular page one'), findsNothing);
expect(find.text('regular page'), findsNothing);
navigatorKey.currentState!.pop();
await tester.pumpAndSettle();
expect(find.text('non-regular page two'), findsNothing);
expect(find.text('non-regular page one'), findsOneWidget);
expect(find.text('regular page'), findsNothing);
});
testWidgets('WidgetsApp.router works', (WidgetTester tester) async {
final PlatformRouteInformationProvider provider = PlatformRouteInformationProvider(
initialRouteInformation: RouteInformation(
uri: Uri.parse('initial'),
),
);
addTearDown(provider.dispose);
final SimpleNavigatorRouterDelegate delegate = SimpleNavigatorRouterDelegate(
builder: (BuildContext context, RouteInformation information) {
return Text(information.uri.toString());
},
onPopPage: (Route<void> route, void result, SimpleNavigatorRouterDelegate delegate) {
delegate.routeInformation = RouteInformation(
uri: Uri.parse('popped'),
);
return route.didPop(result);
},
);
addTearDown(delegate.dispose);
await tester.pumpWidget(WidgetsApp.router(
routeInformationProvider: provider,
routeInformationParser: SimpleRouteInformationParser(),
routerDelegate: delegate,
color: const Color(0xFF123456),
));
expect(find.text('initial'), findsOneWidget);
// Simulate android back button intent.
final ByteData message = const JSONMethodCodec().encodeMethodCall(const MethodCall('popRoute'));
await tester.binding.defaultBinaryMessenger.handlePlatformMessage('flutter/navigation', message, (_) { });
await tester.pumpAndSettle();
expect(find.text('popped'), findsOneWidget);
});
testWidgets('WidgetsApp.router route information parser is optional', (WidgetTester tester) async {
final SimpleNavigatorRouterDelegate delegate = SimpleNavigatorRouterDelegate(
builder: (BuildContext context, RouteInformation information) {
return Text(information.uri.toString());
},
onPopPage: (Route<void> route, void result, SimpleNavigatorRouterDelegate delegate) {
delegate.routeInformation = RouteInformation(
uri: Uri.parse('popped'),
);
return route.didPop(result);
},
);
addTearDown(delegate.dispose);
delegate.routeInformation = RouteInformation(uri: Uri.parse('initial'));
await tester.pumpWidget(WidgetsApp.router(
routerDelegate: delegate,
color: const Color(0xFF123456),
));
expect(find.text('initial'), findsOneWidget);
// Simulate android back button intent.
final ByteData message = const JSONMethodCodec().encodeMethodCall(const MethodCall('popRoute'));
await tester.binding.defaultBinaryMessenger.handlePlatformMessage('flutter/navigation', message, (_) { });
await tester.pumpAndSettle();
expect(find.text('popped'), findsOneWidget);
});
testWidgets('WidgetsApp.router throw if route information provider is provided but no route information parser', (WidgetTester tester) async {
final SimpleNavigatorRouterDelegate delegate = SimpleNavigatorRouterDelegate(
builder: (BuildContext context, RouteInformation information) {
return Text(information.uri.toString());
},
onPopPage: (Route<void> route, void result, SimpleNavigatorRouterDelegate delegate) {
delegate.routeInformation = RouteInformation(
uri: Uri.parse('popped'),
);
return route.didPop(result);
},
);
addTearDown(delegate.dispose);
delegate.routeInformation = RouteInformation(uri: Uri.parse('initial'));
final PlatformRouteInformationProvider provider = PlatformRouteInformationProvider(
initialRouteInformation: RouteInformation(
uri: Uri.parse('initial'),
),
);
addTearDown(provider.dispose);
await expectLater(() async {
await tester.pumpWidget(WidgetsApp.router(
routeInformationProvider: provider,
routerDelegate: delegate,
color: const Color(0xFF123456),
));
}, throwsAssertionError);
});
testWidgets('WidgetsApp.router throw if route configuration is provided along with other delegate', (WidgetTester tester) async {
final SimpleNavigatorRouterDelegate delegate = SimpleNavigatorRouterDelegate(
builder: (BuildContext context, RouteInformation information) {
return Text(information.uri.toString());
},
onPopPage: (Route<void> route, void result, SimpleNavigatorRouterDelegate delegate) {
delegate.routeInformation = RouteInformation(
uri: Uri.parse('popped'),
);
return route.didPop(result);
},
);
addTearDown(delegate.dispose);
delegate.routeInformation = RouteInformation(uri: Uri.parse('initial'));
final RouterConfig<RouteInformation> routerConfig = RouterConfig<RouteInformation>(routerDelegate: delegate);
await expectLater(() async {
await tester.pumpWidget(WidgetsApp.router(
routerDelegate: delegate,
routerConfig: routerConfig,
color: const Color(0xFF123456),
));
}, throwsAssertionError);
});
testWidgets('WidgetsApp.router router config works', (WidgetTester tester) async {
final SimpleNavigatorRouterDelegate delegate = SimpleNavigatorRouterDelegate(
builder: (BuildContext context, RouteInformation information) {
return Text(information.uri.toString());
},
onPopPage: (Route<void> route, void result, SimpleNavigatorRouterDelegate delegate) {
delegate.routeInformation = RouteInformation(
uri: Uri.parse('popped'),
);
return route.didPop(result);
},
);
addTearDown(delegate.dispose);
final PlatformRouteInformationProvider provider = PlatformRouteInformationProvider(
initialRouteInformation: RouteInformation(
uri: Uri.parse('initial'),
),
);
addTearDown(provider.dispose);
final RouterConfig<RouteInformation> routerConfig = RouterConfig<RouteInformation>(
routeInformationProvider: provider,
routeInformationParser: SimpleRouteInformationParser(),
routerDelegate: delegate,
backButtonDispatcher: RootBackButtonDispatcher()
);
await tester.pumpWidget(WidgetsApp.router(
routerConfig: routerConfig,
color: const Color(0xFF123456),
));
expect(find.text('initial'), findsOneWidget);
// Simulate android back button intent.
final ByteData message = const JSONMethodCodec().encodeMethodCall(const MethodCall('popRoute'));
await tester.binding.defaultBinaryMessenger.handlePlatformMessage('flutter/navigation', message, (_) { });
await tester.pumpAndSettle();
expect(find.text('popped'), findsOneWidget);
});
testWidgets('WidgetsApp.router has correct default', (WidgetTester tester) async {
final SimpleNavigatorRouterDelegate delegate = SimpleNavigatorRouterDelegate(
builder: (BuildContext context, RouteInformation information) {
return Text(information.uri.toString());
},
onPopPage: (Route<Object?> route, Object? result, SimpleNavigatorRouterDelegate delegate) => true,
);
addTearDown(delegate.dispose);
await tester.pumpWidget(WidgetsApp.router(
routeInformationParser: SimpleRouteInformationParser(),
routerDelegate: delegate,
color: const Color(0xFF123456),
));
expect(find.text('/'), findsOneWidget);
});
testWidgets('WidgetsApp has correct default ScrollBehavior', (WidgetTester tester) async {
late BuildContext capturedContext;
await tester.pumpWidget(
WidgetsApp(
builder: (BuildContext context, Widget? child) {
capturedContext = context;
return const Placeholder();
},
color: const Color(0xFF123456),
),
);
expect(ScrollConfiguration.of(capturedContext).runtimeType, ScrollBehavior);
});
test('basicLocaleListResolution', () {
// Matches exactly for language code.
expect(
basicLocaleListResolution(
<Locale>[
const Locale('zh'),
const Locale('un'),
const Locale('en'),
],
<Locale>[
const Locale('en'),
],
),
const Locale('en'),
);
// Matches exactly for language code and country code.
expect(
basicLocaleListResolution(
<Locale>[
const Locale('en'),
const Locale('en', 'US'),
],
<Locale>[
const Locale('en', 'US'),
],
),
const Locale('en', 'US'),
);
// Matches language+script over language+country
expect(
basicLocaleListResolution(
<Locale>[
const Locale.fromSubtags(
languageCode: 'zh',
scriptCode: 'Hant',
countryCode: 'HK',
),
],
<Locale>[
const Locale.fromSubtags(
languageCode: 'zh',
countryCode: 'HK',
),
const Locale.fromSubtags(
languageCode: 'zh',
scriptCode: 'Hant',
),
],
),
const Locale.fromSubtags(
languageCode: 'zh',
scriptCode: 'Hant',
),
);
// Matches exactly for language code, script code and country code.
expect(
basicLocaleListResolution(
<Locale>[
const Locale.fromSubtags(
languageCode: 'zh',
),
const Locale.fromSubtags(
languageCode: 'zh',
scriptCode: 'Hant',
countryCode: 'TW',
),
],
<Locale>[
const Locale.fromSubtags(
languageCode: 'zh',
scriptCode: 'Hant',
countryCode: 'TW',
),
],
),
const Locale.fromSubtags(
languageCode: 'zh',
scriptCode: 'Hant',
countryCode: 'TW',
),
);
// Selects for country code if the language code is not found in the
// preferred locales list.
expect(
basicLocaleListResolution(
<Locale>[
const Locale.fromSubtags(
languageCode: 'en',
),
const Locale.fromSubtags(
languageCode: 'ar',
countryCode: 'tn',
),
],
<Locale>[
const Locale.fromSubtags(
languageCode: 'fr',
countryCode: 'tn',
),
],
),
const Locale.fromSubtags(
languageCode: 'fr',
countryCode: 'tn',
),
);
// Selects first (default) locale when no match at all is found.
expect(
basicLocaleListResolution(
<Locale>[
const Locale('tn'),
],
<Locale>[
const Locale('zh'),
const Locale('un'),
const Locale('en'),
],
),
const Locale('zh'),
);
});
testWidgets("WidgetsApp reports an exception if the selected locale isn't supported", (WidgetTester tester) async {
late final List<Locale>? localesArg;
late final Iterable<Locale> supportedLocalesArg;
await tester.pumpWidget(
MaterialApp( // This uses a MaterialApp because it introduces some actual localizations.
localeListResolutionCallback: (List<Locale>? locales, Iterable<Locale> supportedLocales) {
localesArg = locales;
supportedLocalesArg = supportedLocales;
return const Locale('C_UTF-8');
},
builder: (BuildContext context, Widget? child) => const Placeholder(),
color: const Color(0xFF000000),
),
);
if (!kIsWeb) {
// On web, `flutter test` does not guarantee a particular locale, but
// when using `flutter_tester`, we guarantee that it's en-US, zh-CN.
// https://github.com/flutter/flutter/issues/93290
expect(localesArg, const <Locale>[Locale('en', 'US'), Locale('zh', 'CN')]);
}
expect(supportedLocalesArg, const <Locale>[Locale('en', 'US')]);
expect(tester.takeException(), "Warning: This application's locale, C_UTF-8, is not supported by all of its localization delegates.");
});
testWidgets("WidgetsApp doesn't have dependency on MediaQuery", (WidgetTester tester) async {
int routeBuildCount = 0;
final Widget widget = WidgetsApp(
color: const Color.fromARGB(255, 255, 255, 255),
onGenerateRoute: (_) {
return PageRouteBuilder<void>(pageBuilder: (_, __, ___) {
routeBuildCount++;
return const Placeholder();
});
},
);
await tester.pumpWidget(
MediaQuery(data: const MediaQueryData(textScaler: TextScaler.linear(10)), child: widget),
);
expect(routeBuildCount, equals(1));
await tester.pumpWidget(
MediaQuery(data: const MediaQueryData(textScaler: TextScaler.linear(20)), child: widget),
);
expect(routeBuildCount, equals(1));
});
testWidgets('WidgetsApp provides meta based shortcuts for iOS and macOS', (WidgetTester tester) async {
final FocusNode focusNode = FocusNode();
addTearDown(focusNode.dispose);
final SelectAllSpy selectAllSpy = SelectAllSpy();
final CopySpy copySpy = CopySpy();
final PasteSpy pasteSpy = PasteSpy();
final Map<Type, Action<Intent>> actions = <Type, Action<Intent>>{
// Copy Paste
SelectAllTextIntent: selectAllSpy,
CopySelectionTextIntent: copySpy,
PasteTextIntent: pasteSpy,
};
await tester.pumpWidget(
WidgetsApp(
builder: (BuildContext context, Widget? child) {
return Actions(
actions: actions,
child: Focus(
focusNode: focusNode,
child: const Placeholder(),
),
);
},
color: const Color(0xFF123456),
),
);
focusNode.requestFocus();
await tester.pump();
expect(selectAllSpy.invoked, isFalse);
expect(copySpy.invoked, isFalse);
expect(pasteSpy.invoked, isFalse);
// Select all.
await tester.sendKeyDownEvent(LogicalKeyboardKey.metaLeft);
await tester.sendKeyDownEvent(LogicalKeyboardKey.keyA);
await tester.sendKeyUpEvent(LogicalKeyboardKey.keyA);
await tester.sendKeyUpEvent(LogicalKeyboardKey.metaLeft);
await tester.pump();
expect(selectAllSpy.invoked, isTrue);
expect(copySpy.invoked, isFalse);
expect(pasteSpy.invoked, isFalse);
// Copy.
await tester.sendKeyDownEvent(LogicalKeyboardKey.metaLeft);
await tester.sendKeyDownEvent(LogicalKeyboardKey.keyC);
await tester.sendKeyUpEvent(LogicalKeyboardKey.keyC);
await tester.sendKeyUpEvent(LogicalKeyboardKey.metaLeft);
await tester.pump();
expect(selectAllSpy.invoked, isTrue);
expect(copySpy.invoked, isTrue);
expect(pasteSpy.invoked, isFalse);
// Paste.
await tester.sendKeyDownEvent(LogicalKeyboardKey.metaLeft);
await tester.sendKeyDownEvent(LogicalKeyboardKey.keyV);
await tester.sendKeyUpEvent(LogicalKeyboardKey.keyV);
await tester.sendKeyUpEvent(LogicalKeyboardKey.metaLeft);
await tester.pump();
expect(selectAllSpy.invoked, isTrue);
expect(copySpy.invoked, isTrue);
expect(pasteSpy.invoked, isTrue);
}, variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.iOS, TargetPlatform.macOS }));
group('Android Predictive Back', () {
Future<void> setAppLifeCycleState(AppLifecycleState state) async {
final ByteData? message = const StringCodec().encodeMessage(state.toString());
await TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger
.handlePlatformMessage('flutter/lifecycle', message, (ByteData? data) {});
}
final List<bool> frameworkHandlesBacks = <bool>[];
setUp(() async {
frameworkHandlesBacks.clear();
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger
.setMockMethodCallHandler(SystemChannels.platform, (MethodCall methodCall) async {
if (methodCall.method == 'SystemNavigator.setFrameworkHandlesBack') {
expect(methodCall.arguments, isA<bool>());
frameworkHandlesBacks.add(methodCall.arguments as bool);
}
return;
});
});
tearDown(() async {
TestDefaultBinaryMessengerBinding.instance.defaultBinaryMessenger
.setMockMethodCallHandler(SystemChannels.platform, null);
await setAppLifeCycleState(AppLifecycleState.resumed);
});
testWidgets('WidgetsApp calls setFrameworkHandlesBack only when app is ready', (WidgetTester tester) async {
// Start in the `resumed` state, where setFrameworkHandlesBack should be
// called like normal.
await setAppLifeCycleState(AppLifecycleState.resumed);
late BuildContext currentContext;
await tester.pumpWidget(
WidgetsApp(
color: const Color(0xFF123456),
builder: (BuildContext context, Widget? child) {
currentContext = context;
return const Placeholder();
},
),
);
expect(frameworkHandlesBacks, isEmpty);
const NavigationNotification(canHandlePop: true).dispatch(currentContext);
await tester.pumpAndSettle();
expect(frameworkHandlesBacks, isNotEmpty);
expect(frameworkHandlesBacks.last, isTrue);
const NavigationNotification(canHandlePop: false).dispatch(currentContext);
await tester.pumpAndSettle();
expect(frameworkHandlesBacks.last, isFalse);
// Set the app state to inactive, where setFrameworkHandlesBack shouldn't
// be called.
await setAppLifeCycleState(AppLifecycleState.inactive);
final int finalCallsLength = frameworkHandlesBacks.length;
const NavigationNotification(canHandlePop: true).dispatch(currentContext);
await tester.pumpAndSettle();
expect(frameworkHandlesBacks, hasLength(finalCallsLength));
const NavigationNotification(canHandlePop: false).dispatch(currentContext);
await tester.pumpAndSettle();
expect(frameworkHandlesBacks, hasLength(finalCallsLength));
// Set the app state to detached, which also shouldn't call
// setFrameworkHandlesBack. Must go to paused, then detached.
await setAppLifeCycleState(AppLifecycleState.paused);
await setAppLifeCycleState(AppLifecycleState.detached);
const NavigationNotification(canHandlePop: true).dispatch(currentContext);
await tester.pumpAndSettle();
expect(frameworkHandlesBacks, hasLength(finalCallsLength));
const NavigationNotification(canHandlePop: false).dispatch(currentContext);
await tester.pumpAndSettle();
expect(frameworkHandlesBacks, hasLength(finalCallsLength));
},
skip: kIsWeb, // [intended] predictive back is only native Android.
variant: const TargetPlatformVariant(<TargetPlatform>{ TargetPlatform.android })
);
});
}
typedef SimpleRouterDelegateBuilder = Widget Function(BuildContext context, RouteInformation information);
typedef SimpleNavigatorRouterDelegatePopPage<T> = bool Function(Route<T> route, T result, SimpleNavigatorRouterDelegate delegate);
class SelectAllSpy extends Action<SelectAllTextIntent> {
bool invoked = false;
@override
void invoke(SelectAllTextIntent intent) {
invoked = true;
}
}
class CopySpy extends Action<CopySelectionTextIntent> {
bool invoked = false;
@override
void invoke(CopySelectionTextIntent intent) {
invoked = true;
}
}
class PasteSpy extends Action<PasteTextIntent> {
bool invoked = false;
@override
void invoke(PasteTextIntent intent) {
invoked = true;
}
}
class SimpleRouteInformationParser extends RouteInformationParser<RouteInformation> {
SimpleRouteInformationParser();
@override
Future<RouteInformation> parseRouteInformation(RouteInformation information) {
return SynchronousFuture<RouteInformation>(information);
}
@override
RouteInformation restoreRouteInformation(RouteInformation configuration) {
return configuration;
}
}
class SimpleNavigatorRouterDelegate extends RouterDelegate<RouteInformation> with PopNavigatorRouterDelegateMixin<RouteInformation>, ChangeNotifier {
SimpleNavigatorRouterDelegate({
required this.builder,
required this.onPopPage,
});
@override
GlobalKey<NavigatorState> navigatorKey = GlobalKey<NavigatorState>();
RouteInformation get routeInformation => _routeInformation;
late RouteInformation _routeInformation;
set routeInformation(RouteInformation newValue) {
_routeInformation = newValue;
notifyListeners();
}
final SimpleRouterDelegateBuilder builder;
final SimpleNavigatorRouterDelegatePopPage<void> onPopPage;
@override
Future<void> setNewRoutePath(RouteInformation configuration) {
_routeInformation = configuration;
return SynchronousFuture<void>(null);
}
bool _handlePopPage(Route<void> route, void data) {
return onPopPage(route, data, this);
}
@override
Widget build(BuildContext context) {
return Navigator(
key: navigatorKey,
onPopPage: _handlePopPage,
pages: <Page<void>>[
// We need at least two pages for the pop to propagate through.
// Otherwise, the navigator will bubble the pop to the system navigator.
const MaterialPage<void>(
child: Text('base'),
),
MaterialPage<void>(
key: ValueKey<String>(routeInformation.uri.toString()),
child: builder(context, routeInformation),
),
],
);
}
}
| flutter/packages/flutter/test/widgets/app_test.dart/0 | {
"file_path": "flutter/packages/flutter/test/widgets/app_test.dart",
"repo_id": "flutter",
"token_count": 11813
} | 711 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.