text
stringlengths 6
13.6M
| id
stringlengths 13
176
| metadata
dict | __index_level_0__
int64 0
1.69k
|
---|---|---|---|
export 'app_back_button.dart';
export 'app_button.dart';
export 'app_email_text_field.dart';
export 'app_logo.dart';
export 'app_switch.dart';
export 'app_text_field.dart';
export 'content_theme_override_builder.dart';
export 'show_app_modal.dart';
| news_toolkit/flutter_news_example/packages/app_ui/lib/src/widgets/widgets.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/app_ui/lib/src/widgets/widgets.dart",
"repo_id": "news_toolkit",
"token_count": 97
} | 912 |
# article_repository
[![style: very good analysis][very_good_analysis_badge]][very_good_analysis_link]
[![License: MIT][license_badge]][license_link]
A repository that manages article data.
[license_badge]: https://img.shields.io/badge/license-MIT-blue.svg
[license_link]: https://opensource.org/licenses/MIT
[very_good_analysis_badge]: https://img.shields.io/badge/style-very_good_analysis-B22C89.svg
[very_good_analysis_link]: https://pub.dev/packages/very_good_analysis | news_toolkit/flutter_news_example/packages/article_repository/README.md/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/article_repository/README.md",
"repo_id": "news_toolkit",
"token_count": 168
} | 913 |
// This package is just an abstraction.
// See firebase_authentication_client for a concrete implementation
// ignore_for_file: prefer_const_constructors
import 'package:authentication_client/authentication_client.dart';
import 'package:test/fake.dart';
import 'package:test/test.dart';
// AuthenticationClient is exported and can be implemented
class FakeAuthenticationClient extends Fake implements AuthenticationClient {}
void main() {
test('AuthenticationClient can be implemented', () {
expect(FakeAuthenticationClient.new, returnsNormally);
});
test('exports SendLoginEmailLinkFailure', () {
expect(
() => SendLoginEmailLinkFailure('oops'),
returnsNormally,
);
});
test('exports IsLogInWithEmailLinkFailure', () {
expect(
() => IsLogInWithEmailLinkFailure('oops'),
returnsNormally,
);
});
test('exports LogInWithEmailLinkFailure', () {
expect(
() => LogInWithEmailLinkFailure('oops'),
returnsNormally,
);
});
test('exports LogInWithAppleFailure', () {
expect(
() => LogInWithAppleFailure('oops'),
returnsNormally,
);
});
test('exports LogInWithGoogleFailure', () {
expect(
() => LogInWithGoogleFailure('oops'),
returnsNormally,
);
});
test('exports LogInWithGoogleCanceled', () {
expect(
() => LogInWithGoogleCanceled('oops'),
returnsNormally,
);
});
test('exports LogInWithFacebookFailure', () {
expect(
() => LogInWithFacebookFailure('oops'),
returnsNormally,
);
});
test('exports LogInWithFacebookCanceled', () {
expect(
() => LogInWithFacebookCanceled('oops'),
returnsNormally,
);
});
test('exports LogInWithTwitterFailure', () {
expect(
() => LogInWithTwitterFailure('oops'),
returnsNormally,
);
});
test('exports LogInWithTwitterCanceled', () {
expect(
() => LogInWithTwitterCanceled('oops'),
returnsNormally,
);
});
test('exports LogOutFailure', () {
expect(
() => LogOutFailure('oops'),
returnsNormally,
);
});
}
| news_toolkit/flutter_news_example/packages/authentication_client/authentication_client/test/src/authentication_client_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/authentication_client/authentication_client/test/src/authentication_client_test.dart",
"repo_id": "news_toolkit",
"token_count": 741
} | 914 |
include: package:very_good_analysis/analysis_options.5.1.0.yaml
| news_toolkit/flutter_news_example/packages/deep_link_client/analysis_options.yaml/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/deep_link_client/analysis_options.yaml",
"repo_id": "news_toolkit",
"token_count": 23
} | 915 |
import 'package:app_ui/app_ui.dart';
import 'package:flutter/material.dart';
import 'package:news_blocks_ui/src/newsletter/index.dart';
/// {@template newsletter_success}
/// A reusable newsletter success news block widget.
/// {@endtemplate}
class NewsletterSucceeded extends StatelessWidget {
/// {@macro newsletter_success}
const NewsletterSucceeded({
required this.headerText,
required this.content,
required this.footerText,
super.key,
});
/// The header displayed message.
final String headerText;
/// The widget displayed in a center of [NewsletterSucceeded] view.
final Widget content;
/// The footer displayed message.
final String footerText;
@override
Widget build(BuildContext context) {
final theme = Theme.of(context);
return NewsletterContainer(
child: Column(
children: [
Text(
headerText,
textAlign: TextAlign.center,
style: theme.textTheme.headlineMedium?.copyWith(
color: AppColors.highEmphasisPrimary,
),
),
const SizedBox(height: AppSpacing.lg + AppSpacing.lg),
content,
const SizedBox(height: AppSpacing.lg + AppSpacing.lg),
Text(
footerText,
textAlign: TextAlign.center,
style: theme.textTheme.bodyLarge?.copyWith(
color: AppColors.mediumHighEmphasisPrimary,
),
),
],
),
);
}
}
| news_toolkit/flutter_news_example/packages/news_blocks_ui/lib/src/newsletter/newsletter_succeeded.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/news_blocks_ui/lib/src/newsletter/newsletter_succeeded.dart",
"repo_id": "news_toolkit",
"token_count": 617
} | 916 |
import 'package:app_ui/app_ui.dart';
import 'package:flutter/material.dart';
import 'package:news_blocks/news_blocks.dart';
/// {@template text_headline}
/// A reusable text headline news block widget.
/// {@endtemplate}
class TextHeadline extends StatelessWidget {
/// {@macro text_headline}
const TextHeadline({required this.block, super.key});
/// The associated [TextHeadlineBlock] instance.
final TextHeadlineBlock block;
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.symmetric(horizontal: AppSpacing.lg),
child: Text(
block.text,
style: Theme.of(context).textTheme.displayMedium,
),
);
}
}
| news_toolkit/flutter_news_example/packages/news_blocks_ui/lib/src/text_headline.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/news_blocks_ui/lib/src/text_headline.dart",
"repo_id": "news_toolkit",
"token_count": 245
} | 917 |
import 'package:app_ui/app_ui.dart';
import 'package:flutter/material.dart';
/// {@template post_footer}
/// A reusable footer of a post news block widget.
/// {@endtemplate}
class PostFooter extends StatelessWidget {
/// {@macro post_footer}
const PostFooter({
super.key,
this.publishedAt,
this.author,
this.onShare,
this.isContentOverlaid = false,
});
/// The author of this post.
final String? author;
/// The date when this post was published.
final DateTime? publishedAt;
/// Called when the share button is tapped.
final VoidCallback? onShare;
/// Whether footer is displayed in reversed color theme.
///
/// Defaults to false.
final bool isContentOverlaid;
@override
Widget build(BuildContext context) {
final textTheme = Theme.of(context).textTheme;
final textColor = isContentOverlaid
? AppColors.mediumHighEmphasisPrimary
: AppColors.mediumEmphasisSurface;
return Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
RichText(
text: TextSpan(
style: textTheme.bodySmall?.copyWith(color: textColor),
children: <InlineSpan>[
if (author != null)
TextSpan(
text: author,
),
if (author != null && publishedAt != null) ...[
const WidgetSpan(child: SizedBox(width: AppSpacing.sm)),
const TextSpan(
text: '•',
),
const WidgetSpan(child: SizedBox(width: AppSpacing.sm)),
],
if (publishedAt != null)
TextSpan(
text: publishedAt!.mDY,
),
],
),
),
if (onShare != null)
IconButton(
icon: Icon(
Icons.share,
color: textColor,
),
onPressed: onShare,
),
],
);
}
}
| news_toolkit/flutter_news_example/packages/news_blocks_ui/lib/src/widgets/post_footer.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/news_blocks_ui/lib/src/widgets/post_footer.dart",
"repo_id": "news_toolkit",
"token_count": 940
} | 918 |
// ignore_for_file: prefer_const_constructors
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:news_blocks_ui/src/sliver_grid_custom_delegate.dart';
void main() {
group('HeaderGridTileLayout', () {
testWidgets(
'getMinChildIndexForScrollOffset when '
'super.getMinChildIndexForScrollOffset is 0', (tester) async {
final headerTileLayout = HeaderGridTileLayout(
crossAxisCount: 1,
mainAxisStride: 14,
crossAxisStride: 15,
childMainAxisExtent: 2,
childCrossAxisExtent: 3,
reverseCrossAxis: false,
);
final result = headerTileLayout.getMinChildIndexForScrollOffset(0);
expect(result, 0);
});
testWidgets(
'getMinChildIndexForScrollOffset when '
'super.getMinChildIndexForScrollOffset is greater than 0',
(tester) async {
final headerTileLayout = HeaderGridTileLayout(
crossAxisCount: 1,
mainAxisStride: 14,
crossAxisStride: 15,
childMainAxisExtent: 2,
childCrossAxisExtent: 3,
reverseCrossAxis: false,
);
final result = headerTileLayout.getMinChildIndexForScrollOffset(100);
expect(result, 6);
});
testWidgets(
'getGeometryForChildIndex when index is equal to 0 (first element) ',
(tester) async {
final headerTileLayout = HeaderGridTileLayout(
crossAxisCount: 1,
mainAxisStride: 14,
crossAxisStride: 15,
childMainAxisExtent: 2,
childCrossAxisExtent: 3,
reverseCrossAxis: false,
);
final geometry = headerTileLayout.getGeometryForChildIndex(0);
final expectedGeometry = SliverGridGeometry(
scrollOffset: 0,
crossAxisOffset: 0,
mainAxisExtent: 16,
crossAxisExtent: 18,
);
expect(geometry.crossAxisExtent, expectedGeometry.crossAxisExtent);
expect(geometry.scrollOffset, expectedGeometry.scrollOffset);
expect(geometry.mainAxisExtent, expectedGeometry.mainAxisExtent);
expect(geometry.crossAxisExtent, expectedGeometry.crossAxisExtent);
});
testWidgets('getGeometryForChildIndex when index is not equal to 0',
(tester) async {
final headerTileLayout = HeaderGridTileLayout(
crossAxisCount: 1,
mainAxisStride: 14,
crossAxisStride: 15,
childMainAxisExtent: 2,
childCrossAxisExtent: 3,
reverseCrossAxis: false,
);
final geometry = headerTileLayout.getGeometryForChildIndex(2);
final expectedGeometry = SliverGridGeometry(
scrollOffset: 44,
crossAxisOffset: 0,
mainAxisExtent: 14,
crossAxisExtent: 3,
);
expect(geometry.crossAxisExtent, expectedGeometry.crossAxisExtent);
expect(geometry.scrollOffset, expectedGeometry.scrollOffset);
expect(geometry.mainAxisExtent, expectedGeometry.mainAxisExtent);
expect(geometry.crossAxisExtent, expectedGeometry.crossAxisExtent);
});
testWidgets('computeMaxScrollOffset', (tester) async {
final headerTileLayout = HeaderGridTileLayout(
crossAxisCount: 1,
mainAxisStride: 14,
crossAxisStride: 15,
childMainAxisExtent: 2,
childCrossAxisExtent: 3,
reverseCrossAxis: false,
);
final maxScrollOffset = headerTileLayout.computeMaxScrollOffset(2);
expect(maxScrollOffset, 58);
});
});
}
| news_toolkit/flutter_news_example/packages/news_blocks_ui/test/src/header_grid_tile_layout_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/news_blocks_ui/test/src/header_grid_tile_layout_test.dart",
"repo_id": "news_toolkit",
"token_count": 1463
} | 919 |
// ignore_for_file: prefer_const_constructors
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:mocktail_image_network/mocktail_image_network.dart';
import 'package:news_blocks/news_blocks.dart';
import 'package:news_blocks_ui/news_blocks_ui.dart';
import '../helpers/helpers.dart';
void main() {
group('PostSmall', () {
setUpAll(() {
setUpTolerantComparator();
setUpMockPathProvider();
});
testWidgets('renders correctly without image', (tester) async {
await mockNetworkImages(() async {
await tester.pumpApp(
Column(
children: [
PostSmall(
block: PostSmallBlock(
id: 'id',
category: PostCategory.technology,
publishedAt: DateTime(2022, 03, 12),
title: 'Nvidia and AMD GPUs are '
'returning to shelves and prices '
'are finally falling',
author: 'Sean Hollister',
),
),
],
),
);
await expectLater(
find.byType(PostSmall),
matchesGoldenFile('post_small_without_image.png'),
);
});
});
testWidgets('renders correctly with image', (tester) async {
await mockNetworkImages(() async {
await tester.pumpApp(
Column(
children: [
PostSmall(
block: PostSmallBlock(
id: 'id',
category: PostCategory.technology,
publishedAt: DateTime(2022, 03, 12),
title: 'Nvidia and AMD GPUs are '
'returning to shelves and prices '
'are finally falling',
author: 'Sean Hollister',
imageUrl:
'https://cdn.vox-cdn.com/thumbor/OTpmptgr7XcTVAJ27UBvIxl0vrg=/0x146:2040x1214/fit-in/1200x630/cdn.vox-cdn.com/uploads/chorus_asset/file/22049166/shollister_201117_4303_0003.0.jpg',
),
),
],
),
);
await expectLater(
find.byType(PostSmall),
matchesGoldenFile('post_small_with_image.png'),
);
});
});
testWidgets('onPressed is called with action when tapped', (tester) async {
final action = NavigateToArticleAction(articleId: 'id');
final actions = <BlockAction>[];
await mockNetworkImages(() async {
await tester.pumpApp(
Column(
children: [
PostSmall(
block: PostSmallBlock(
id: 'id',
category: PostCategory.technology,
publishedAt: DateTime(2022, 03, 12),
title:
'Nvidia and AMD GPUs are returning to shelves and prices '
'are finally falling',
author: 'Sean Hollister',
imageUrl:
'https://cdn.vox-cdn.com/thumbor/OTpmptgr7XcTVAJ27UBvIxl0vrg=/0x146:2040x1214/fit-in/1200x630/cdn.vox-cdn.com/uploads/chorus_asset/file/22049166/shollister_201117_4303_0003.0.jpg',
action: action,
),
onPressed: actions.add,
),
],
),
);
});
await tester.ensureVisible(find.byType(PostSmall));
await tester.tap(find.byType(PostSmall));
expect(actions, equals([action]));
});
});
}
| news_toolkit/flutter_news_example/packages/news_blocks_ui/test/src/post_small_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/news_blocks_ui/test/src/post_small_test.dart",
"repo_id": "news_toolkit",
"token_count": 1871
} | 920 |
// ignore_for_file: unnecessary_const, prefer_const_constructors
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:mocktail_image_network/mocktail_image_network.dart';
import 'package:news_blocks/news_blocks.dart';
import 'package:news_blocks_ui/news_blocks_ui.dart';
import 'package:news_blocks_ui/src/widgets/widgets.dart';
import 'package:video_player_platform_interface/video_player_platform_interface.dart';
import '../helpers/helpers.dart';
void main() {
const category = PostCategory.technology;
const videoUrl =
'https://cdn.vox-cdn.com/thumbor/OTpmptgr7XcTVAJ27UBvIxl0vrg='
'/0x146:2040x1214/fit-in/1200x630/cdn.vox-cdn.com/uploads/chorus_asset'
'/file/22049166/shollister_201117_4303_0003.0.jpg';
const title = 'Nvidia and AMD GPUs are returning to shelves '
'and prices are finally falling';
group('VideoIntroduction', () {
setUpAll(
() {
final fakeVideoPlayerPlatform = FakeVideoPlayerPlatform();
VideoPlayerPlatform.instance = fakeVideoPlayerPlatform;
setUpTolerantComparator();
},
);
testWidgets('renders correctly', (tester) async {
final technologyVideoIntroduction = VideoIntroductionBlock(
category: category,
videoUrl: videoUrl,
title: title,
);
await mockNetworkImages(
() async => tester.pumpContentThemedApp(
SingleChildScrollView(
child: Column(
children: [
VideoIntroduction(block: technologyVideoIntroduction),
],
),
),
),
);
expect(find.byType(InlineVideo), findsOneWidget);
expect(find.byType(PostContent), findsOneWidget);
});
});
}
| news_toolkit/flutter_news_example/packages/news_blocks_ui/test/src/video_introduction_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/news_blocks_ui/test/src/video_introduction_test.dart",
"repo_id": "news_toolkit",
"token_count": 716
} | 921 |
import 'package:firebase_messaging/firebase_messaging.dart';
import 'package:notifications_client/notifications_client.dart';
/// {@template firebase_notifications_client}
/// A Firebase Cloud Messaging notifications client.
/// {@endtemplate}
class FirebaseNotificationsClient implements NotificationsClient {
/// {@macro firebase_notifications_client}
const FirebaseNotificationsClient({
required FirebaseMessaging firebaseMessaging,
}) : _firebaseMessaging = firebaseMessaging;
final FirebaseMessaging _firebaseMessaging;
/// Subscribes to the notification group based on [category].
@override
Future<void> subscribeToCategory(String category) async {
try {
await _firebaseMessaging.subscribeToTopic(category);
} catch (error, stackTrace) {
Error.throwWithStackTrace(
SubscribeToCategoryFailure(error),
stackTrace,
);
}
}
/// Unsubscribes from the notification group based on [category].
@override
Future<void> unsubscribeFromCategory(String category) async {
try {
await _firebaseMessaging.unsubscribeFromTopic(category);
} catch (error, stackTrace) {
Error.throwWithStackTrace(
UnsubscribeFromCategoryFailure(error),
stackTrace,
);
}
}
}
| news_toolkit/flutter_news_example/packages/notifications_client/firebase_notifications_client/lib/src/firebase_notifications_client.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/notifications_client/firebase_notifications_client/lib/src/firebase_notifications_client.dart",
"repo_id": "news_toolkit",
"token_count": 417
} | 922 |
// ignore_for_file: prefer_const_constructors
import 'package:flutter_test/flutter_test.dart';
import 'package:mocktail/mocktail.dart';
import 'package:notifications_client/notifications_client.dart';
import 'package:one_signal_notifications_client/one_signal_notifications_client.dart';
import 'package:onesignal_flutter/onesignal_flutter.dart';
class MockOneSignal extends Mock implements OneSignal {}
void main() {
group('OneSignalNotificationsClient', () {
late OneSignal oneSignal;
late OneSignalNotificationsClient oneSignalNotificationsClient;
const category = 'category';
setUp(() {
oneSignal = MockOneSignal();
oneSignalNotificationsClient = OneSignalNotificationsClient(
oneSignal: oneSignal,
);
});
group('when OneSignalNotificationsClient.subscribeToCategory called', () {
test('calls OneSignal.sendTag', () async {
when(
() => oneSignal.sendTag(category, true),
).thenAnswer((_) async => {});
await oneSignalNotificationsClient.subscribeToCategory(category);
verify(() => oneSignal.sendTag(category, true)).called(1);
});
test(
'throws SubscribeToCategoryFailure '
'when OneSignal.deleteTag fails', () async {
when(
() => oneSignal.sendTag(category, true),
).thenAnswer((_) async => throw Exception());
expect(
() => oneSignalNotificationsClient.subscribeToCategory(category),
throwsA(isA<SubscribeToCategoryFailure>()),
);
});
});
group('when OneSignalNotificationsClient.unsubscribeFromCategory called',
() {
test('calls OneSignal.deleteTag', () async {
when(() => oneSignal.deleteTag(category)).thenAnswer((_) async => {});
await oneSignalNotificationsClient.unsubscribeFromCategory(category);
verify(() => oneSignal.deleteTag(category)).called(1);
});
test(
'throws UnsubscribeFromCategoryFailure '
'when OneSignal.deleteTag fails', () async {
when(
() => oneSignal.deleteTag(category),
).thenAnswer((_) async => throw Exception());
expect(
() => oneSignalNotificationsClient.unsubscribeFromCategory(category),
throwsA(isA<UnsubscribeFromCategoryFailure>()),
);
});
});
});
}
| news_toolkit/flutter_news_example/packages/notifications_client/one_signal_notifications_client/test/src/one_signal_notifications_client_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/notifications_client/one_signal_notifications_client/test/src/one_signal_notifications_client_test.dart",
"repo_id": "news_toolkit",
"token_count": 918
} | 923 |
// ignore_for_file: prefer_const_constructors
import 'package:package_info_client/package_info_client.dart';
import 'package:test/test.dart';
void main() {
group('PackageInfoClient', () {
test('returns correct package info', () async {
const appName = 'App';
const packageName = 'com.example.app';
const packageVersion = '1.0.0';
final packageInfoClient = PackageInfoClient(
appName: appName,
packageName: packageName,
packageVersion: packageVersion,
);
expect(packageInfoClient.appName, appName);
expect(packageInfoClient.packageName, packageName);
expect(packageInfoClient.packageVersion, packageVersion);
});
});
}
| news_toolkit/flutter_news_example/packages/package_info_client/test/src/package_info_client_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/package_info_client/test/src/package_info_client_test.dart",
"repo_id": "news_toolkit",
"token_count": 253
} | 924 |
export 'src/secure_storage.dart';
| news_toolkit/flutter_news_example/packages/storage/secure_storage/lib/secure_storage.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/storage/secure_storage/lib/secure_storage.dart",
"repo_id": "news_toolkit",
"token_count": 12
} | 925 |
export 'package:authentication_client/authentication_client.dart'
show
AuthenticationException,
IsLogInWithEmailLinkFailure,
LogInWithAppleFailure,
LogInWithEmailLinkFailure,
LogInWithFacebookCanceled,
LogInWithFacebookFailure,
LogInWithGoogleCanceled,
LogInWithGoogleFailure,
LogInWithTwitterCanceled,
LogInWithTwitterFailure,
LogOutFailure,
SendLoginEmailLinkFailure;
export 'src/models/models.dart';
export 'src/user_repository.dart';
| news_toolkit/flutter_news_example/packages/user_repository/lib/user_repository.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/user_repository/lib/user_repository.dart",
"repo_id": "news_toolkit",
"token_count": 222
} | 926 |
// ignore_for_file: must_be_immutable, prefer_const_constructors
import 'package:flutter_news_example/app/app.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:in_app_purchase_repository/in_app_purchase_repository.dart';
import 'package:mocktail/mocktail.dart';
import 'package:user_repository/user_repository.dart';
class MockUser extends Mock implements User {}
void main() {
group('AppState', () {
late User user;
setUp(() {
user = MockUser();
when(() => user.subscriptionPlan).thenReturn(SubscriptionPlan.none);
});
group('unauthenticated', () {
test('has correct status', () {
final state = AppState.unauthenticated();
expect(state.status, AppStatus.unauthenticated);
expect(state.user, User.anonymous);
});
});
group('authenticated', () {
test('has correct status', () {
final state = AppState.authenticated(user);
expect(state.status, AppStatus.authenticated);
expect(state.user, user);
});
});
group('onboardingRequired', () {
test('has correct status', () {
final state = AppState.onboardingRequired(user);
expect(state.status, AppStatus.onboardingRequired);
expect(state.user, user);
});
});
group('isUserSubscribed', () {
test('returns true when userSubscriptionPlan is not null and not none',
() {
when(() => user.subscriptionPlan).thenReturn(SubscriptionPlan.premium);
expect(
AppState.authenticated(user).isUserSubscribed,
isTrue,
);
});
test('returns false when userSubscriptionPlan is none', () {
expect(
AppState.authenticated(user).isUserSubscribed,
isFalse,
);
});
});
group('AppStatus', () {
test(
'authenticated and onboardingRequired are the only statuses '
'where loggedIn is true', () {
expect(
AppStatus.values.where((e) => e.isLoggedIn).toList(),
equals(
[
AppStatus.onboardingRequired,
AppStatus.authenticated,
],
),
);
});
});
group('copyWith', () {
test(
'returns same object '
'when no properties are passed', () {
expect(
AppState.unauthenticated().copyWith(),
equals(AppState.unauthenticated()),
);
});
test(
'returns object with updated status '
'when status is passed', () {
expect(
AppState.unauthenticated().copyWith(
status: AppStatus.onboardingRequired,
),
equals(
AppState(
status: AppStatus.onboardingRequired,
),
),
);
});
test(
'returns object with updated user '
'when user is passed', () {
expect(
AppState.unauthenticated().copyWith(
user: user,
),
equals(
AppState(
status: AppStatus.unauthenticated,
user: user,
),
),
);
});
});
});
}
| news_toolkit/flutter_news_example/test/app/bloc/app_state_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/test/app/bloc/app_state_test.dart",
"repo_id": "news_toolkit",
"token_count": 1463
} | 927 |
// ignore_for_file: prefer_const_constructors
import 'package:flutter_news_example/categories/categories.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:news_blocks/news_blocks.dart';
void main() {
group('CategoriesEvent', () {
group('CategoriesRequested', () {
test('supports value comparisons', () {
final event1 = CategoriesRequested();
final event2 = CategoriesRequested();
expect(event1, equals(event2));
});
});
group('CategorySelected', () {
test('supports value comparisons', () {
final event1 = CategorySelected(category: Category.top);
final event2 = CategorySelected(category: Category.top);
expect(event1, equals(event2));
});
});
});
}
| news_toolkit/flutter_news_example/test/categories/bloc/categories_event_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/test/categories/bloc/categories_event_test.dart",
"repo_id": "news_toolkit",
"token_count": 282
} | 928 |
// ignore_for_file: prefer_const_constructors
// ignore_for_file: prefer_const_literals_to_create_immutables
import 'package:flutter/material.dart';
import 'package:flutter_news_example/feed/feed.dart';
import 'package:flutter_news_example/home/home.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:mocktail/mocktail.dart';
import 'package:news_repository/news_repository.dart';
import '../../helpers/helpers.dart';
class MockNewsRepository extends Mock implements NewsRepository {}
void main() {
initMockHydratedStorage();
late NewsRepository newsRepository;
setUp(() {
newsRepository = MockNewsRepository();
when(newsRepository.getCategories).thenAnswer(
(_) async => CategoriesResponse(
categories: [Category.top],
),
);
});
test('has a page', () {
expect(HomePage.page(), isA<MaterialPage<void>>());
});
testWidgets('renders a HomeView', (tester) async {
await tester.pumpApp(const HomePage());
expect(find.byType(HomeView), findsOneWidget);
});
testWidgets('renders FeedView', (tester) async {
await tester.pumpApp(
const HomePage(),
newsRepository: newsRepository,
);
expect(find.byType(FeedView), findsOneWidget);
});
}
| news_toolkit/flutter_news_example/test/home/view/home_page_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/test/home/view/home_page_test.dart",
"repo_id": "news_toolkit",
"token_count": 447
} | 929 |
import 'package:flutter_news_example/main/build_number.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
group('buildNumber', () {
test('is not null', () {
expect(buildNumber(), isNotNull);
});
test('returns 0 when packageVersion is empty', () {
expect(buildNumber(''), 0);
});
test('returns 0 when packageVersion is missing a build number', () {
expect(buildNumber('1.0.0'), 0);
});
test('returns 0 when packageVersion has a malformed build number', () {
expect(buildNumber('1.0.0+'), 0);
});
test('returns 42 when build number is 42', () {
expect(buildNumber('1.0.0+42'), 42);
});
});
}
| news_toolkit/flutter_news_example/test/main/build_number/build_number_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/test/main/build_number/build_number_test.dart",
"repo_id": "news_toolkit",
"token_count": 261
} | 930 |
// ignore_for_file: prefer_const_constructors
import 'package:flutter_news_example/onboarding/onboarding.dart';
import 'package:test/test.dart';
void main() {
group('OnboardingEvent', () {
group('EnableAdTrackingRequested', () {
test('supports value comparisons', () {
final event1 = EnableAdTrackingRequested();
final event2 = EnableAdTrackingRequested();
expect(event1, equals(event2));
});
});
group('EnableNotificationsRequested', () {
test('supports value comparisons', () {
final event1 = EnableNotificationsRequested();
final event2 = EnableNotificationsRequested();
expect(event1, equals(event2));
});
});
});
}
| news_toolkit/flutter_news_example/test/onboarding/bloc/onboarding_event_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/test/onboarding/bloc/onboarding_event_test.dart",
"repo_id": "news_toolkit",
"token_count": 262
} | 931 |
import 'dart:async';
import 'package:app_ui/app_ui.dart';
import 'package:flutter/material.dart';
import 'package:flutter_news_example/analytics/analytics.dart';
import 'package:flutter_news_example/subscriptions/subscriptions.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:in_app_purchase_repository/in_app_purchase_repository.dart'
hide PurchaseCompleted;
import 'package:mockingjay/mockingjay.dart';
import 'package:user_repository/user_repository.dart';
import '../../../helpers/helpers.dart';
void main() {
late InAppPurchaseRepository inAppPurchaseRepository;
late UserRepository userRepository;
const subscription = Subscription(
id: 'dd339fda-33e9-49d0-9eb5-0ccb77eb760f',
name: SubscriptionPlan.premium,
cost: SubscriptionCost(
annual: 16200,
monthly: 1499,
),
benefits: [
'first',
'second',
'third',
],
);
setUp(() {
inAppPurchaseRepository = MockInAppPurchaseRepository();
userRepository = MockUserRepository();
when(() => inAppPurchaseRepository.purchaseUpdate).thenAnswer(
(_) => const Stream.empty(),
);
when(inAppPurchaseRepository.fetchSubscriptions).thenAnswer(
(_) async => [],
);
when(
userRepository.updateSubscriptionPlan,
).thenAnswer((_) async {});
});
group('showPurchaseSubscriptionDialog', () {
testWidgets('renders PurchaseSubscriptionDialog', (tester) async {
await tester.pumpApp(
Builder(
builder: (context) {
return ElevatedButton(
key: const Key('tester_elevatedButton'),
child: const Text('test'),
onPressed: () => showPurchaseSubscriptionDialog(context: context),
);
},
),
inAppPurchaseRepository: inAppPurchaseRepository,
);
await tester.tap(find.byKey(const Key('tester_elevatedButton')));
await tester.pump();
expect(find.byType(PurchaseSubscriptionDialog), findsOneWidget);
});
});
group('PurchaseSubscriptionDialog', () {
testWidgets(
'renders PurchaseSubscriptionDialogView',
(WidgetTester tester) async {
await tester.pumpApp(
const PurchaseSubscriptionDialog(),
inAppPurchaseRepository: inAppPurchaseRepository,
);
expect(find.byType(PurchaseSubscriptionDialogView), findsOneWidget);
},
);
});
group('PurchaseSubscriptionDialogView', () {
testWidgets('renders list of SubscriptionCard', (tester) async {
const otherSubscription = Subscription(
id: 'other_subscription_id',
name: SubscriptionPlan.premium,
cost: SubscriptionCost(
annual: 16200,
monthly: 1499,
),
benefits: [
'first',
'second',
'third',
],
);
final subscriptions = [
subscription,
otherSubscription,
];
when(inAppPurchaseRepository.fetchSubscriptions).thenAnswer(
(_) async => subscriptions,
);
await tester.pumpApp(
const PurchaseSubscriptionDialog(),
inAppPurchaseRepository: inAppPurchaseRepository,
);
for (final subscription in subscriptions) {
expect(
find.byWidgetPredicate(
(widget) =>
widget is SubscriptionCard &&
widget.key == ValueKey(subscription),
),
findsOneWidget,
);
}
});
testWidgets('closes dialog on close button tap', (tester) async {
final navigator = MockNavigator();
when(navigator.pop).thenAnswer((_) async => true);
await tester.pumpApp(
const PurchaseSubscriptionDialog(),
inAppPurchaseRepository: inAppPurchaseRepository,
navigator: navigator,
);
await tester.pump();
await tester.tap(
find.byKey(
const Key('purchaseSubscriptionDialog_closeIconButton'),
),
);
await tester.pump();
verify(navigator.pop).called(1);
});
testWidgets(
'shows PurchaseCompleted dialog '
'and adds UserSubscriptionConversionEvent to AnalyticsBloc '
'when SubscriptionsBloc emits purchaseStatus.completed',
(tester) async {
final navigator = MockNavigator();
final analyticsBloc = MockAnalyticsBloc();
when(navigator.maybePop<void>).thenAnswer((_) async => true);
when(
() => inAppPurchaseRepository.purchaseUpdate,
).thenAnswer(
(_) => Stream.fromIterable(
[const PurchaseDelivered(subscription: subscription)],
),
);
await tester.pumpApp(
const PurchaseSubscriptionDialog(),
navigator: navigator,
inAppPurchaseRepository: inAppPurchaseRepository,
analyticsBloc: analyticsBloc,
userRepository: userRepository,
);
await tester.pump();
expect(find.byType(PurchaseCompletedDialog), findsOneWidget);
verify(
() => analyticsBloc.add(
TrackAnalyticsEvent(
UserSubscriptionConversionEvent(),
),
),
).called(1);
});
});
group('PurchaseCompletedDialog', () {
testWidgets('closes after 3 seconds', (tester) async {
const buttonText = 'buttonText';
await tester.pumpApp(
Builder(
builder: (context) {
return AppButton.black(
child: const Text('buttonText'),
onPressed: () => showAppModal<void>(
context: context,
builder: (context) => const PurchaseCompletedDialog(),
),
);
},
),
inAppPurchaseRepository: inAppPurchaseRepository,
);
await tester.tap(find.text(buttonText));
await tester.pump();
expect(find.byType(PurchaseCompletedDialog), findsOneWidget);
await tester.pumpAndSettle(const Duration(seconds: 3));
await tester.pump();
expect(find.byType(PurchaseCompletedDialog), findsNothing);
});
});
}
| news_toolkit/flutter_news_example/test/subscriptions/dialog/view/purchase_subscription_dialog_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/test/subscriptions/dialog/view/purchase_subscription_dialog_test.dart",
"repo_id": "news_toolkit",
"token_count": 2548
} | 932 |
#!/bin/bash
# This script can be used to run flutter test for a given directory (defaults to the current directory)
# It will exclude generated code and translations (mimicking the ci) and open the coverage report in a
# new window once it has run successfully.
#
# To run in main project:
# ./tool/coverage.sh
#
# To run in other directory:
# ./tool/coverage.sh ./path/to/other/project
set -e
PROJECT_PATH="${1:-.}"
PROJECT_COVERAGE=./coverage/lcov.info
cd ${PROJECT_PATH}
rm -rf coverage
fvm flutter --version
very_good test --coverage --exclude-coverage "**/*.g.dart **/*.gen.dart **/l10n/*.dart **/l10n/**/*.dart **/main/bootstrap.dart" --exclude-tags 'presubmit-only' --test-randomize-ordering-seed random
lcov --remove ${PROJECT_COVERAGE} -o ${PROJECT_COVERAGE} \
'**/*.g.dart' \
'**/l10n/*.dart' \
'**/l10n/**/*.dart' \
'**/main/bootstrap.dart' \
'**/*.gen.dart'
genhtml ${PROJECT_COVERAGE} -o coverage | tee ./coverage/output.txt
COV_LINE=$(tail -2 ./coverage/output.txt | head -1)
SUB='100.0%'
if [[ "$COV_LINE" == *"$SUB"* ]]; then
echo "The coverage is 100%"
else
echo "Coverage is below 100%! Check the report to see which lines are not covered."
echo $COV_LINE
open ./coverage/index.html
fi
| news_toolkit/flutter_news_example/tool/coverage.sh/0 | {
"file_path": "news_toolkit/flutter_news_example/tool/coverage.sh",
"repo_id": "news_toolkit",
"token_count": 485
} | 933 |
buildscript {
repositories {
google()
mavenCentral()
}
dependencies {
classpath 'com.android.tools.build:gradle:4.1.0'
}
}
allprojects {
repositories {
google()
mavenCentral()
}
}
rootProject.buildDir = '../build'
subprojects {
project.buildDir = "${rootProject.buildDir}/${project.name}"
}
subprojects {
project.evaluationDependsOn(':app')
}
task clean(type: Delete) {
delete rootProject.buildDir
}
| packages/.ci/legacy_project/all_packages/android/build.gradle/0 | {
"file_path": "packages/.ci/legacy_project/all_packages/android/build.gradle",
"repo_id": "packages",
"token_count": 202
} | 934 |
tasks:
- name: prepare tool
script: .ci/scripts/prepare_tool.sh
infra_step: true # Note infra steps failing prevents "always" from running.
- name: download Dart and Android deps
script: .ci/scripts/tool_runner.sh
infra_step: true
args: ["fetch-deps", "--android", "--supporting-target-platforms-only"]
- name: Firebase Test Lab
script: .ci/scripts/tool_runner.sh
args:
- "firebase-test-lab"
- "--device"
- "model=panther,version=33"
- "--exclude=script/configs/exclude_integration_android.yaml"
- "--project=flutter-infra-staging"
- "--results-bucket=flutter_firebase_testlab_staging"
| packages/.ci/targets/android_device_tests.yaml/0 | {
"file_path": "packages/.ci/targets/android_device_tests.yaml",
"repo_id": "packages",
"token_count": 266
} | 935 |
tasks:
- name: prepare tool
script: .ci/scripts/prepare_tool.sh
infra_step: true # Note infra steps failing prevents "always" from running.
- name: create all_packages app
script: .ci/scripts/create_all_packages_app.sh
infra_step: true # Note infra steps failing prevents "always" from running.
# No debug version, unlike the other platforms, since web does not support
# debug builds.
- name: build all_packages app for Web release
script: .ci/scripts/build_all_packages_app.sh
args: ["web", "release"]
| packages/.ci/targets/web_build_all_packages.yaml/0 | {
"file_path": "packages/.ci/targets/web_build_all_packages.yaml",
"repo_id": "packages",
"token_count": 172
} | 936 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:animations/src/open_container.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets(
'Container opens - Fade (by default)',
(WidgetTester tester) async {
const ShapeBorder shape = RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(8.0)),
);
bool closedBuilderCalled = false;
bool openBuilderCalled = false;
await tester.pumpWidget(_boilerplate(
child: Center(
child: OpenContainer(
closedColor: Colors.green,
openColor: Colors.blue,
closedElevation: 4.0,
openElevation: 8.0,
closedShape: shape,
closedBuilder: (BuildContext context, VoidCallback _) {
closedBuilderCalled = true;
return const Text('Closed');
},
openBuilder: (BuildContext context, VoidCallback _) {
openBuilderCalled = true;
return const Text('Open');
},
),
),
));
// Closed container has the expected properties.
final StatefulElement srcMaterialElement = tester.firstElement(
find.ancestor(
of: find.text('Closed'),
matching: find.byType(Material),
),
);
final Material srcMaterial = srcMaterialElement.widget as Material;
expect(srcMaterial.color, Colors.green);
expect(srcMaterial.elevation, 4.0);
expect(srcMaterial.shape, shape);
expect(find.text('Closed'), findsOneWidget);
expect(find.text('Open'), findsNothing);
expect(closedBuilderCalled, isTrue);
expect(openBuilderCalled, isFalse);
final Rect srcMaterialRect = tester.getRect(
find.byElementPredicate((Element e) => e == srcMaterialElement),
);
// Open the container.
await tester.tap(find.text('Closed'));
expect(find.text('Closed'), findsOneWidget);
expect(find.text('Open'), findsNothing);
await tester.pump();
// On the first frame of the animation everything still looks like before.
final StatefulElement destMaterialElement = tester.firstElement(
find.ancestor(
of: find.text('Closed'),
matching: find.byType(Material),
),
);
final Material closedMaterial = destMaterialElement.widget as Material;
expect(closedMaterial.color, Colors.green);
expect(closedMaterial.elevation, 4.0);
expect(closedMaterial.shape, shape);
expect(find.text('Closed'), findsOneWidget);
expect(find.text('Open'), findsOneWidget);
final Rect closedMaterialRect = tester.getRect(
find.byElementPredicate((Element e) => e == destMaterialElement),
);
expect(closedMaterialRect, srcMaterialRect);
expect(_getOpacity(tester, 'Open'), 0.0);
expect(_getOpacity(tester, 'Closed'), 1.0);
final _TrackedData dataClosed = _TrackedData(
closedMaterial,
closedMaterialRect,
);
// Jump to the start of the fade in.
await tester.pump(const Duration(milliseconds: 60)); // 300ms * 1/5 = 60ms
final _TrackedData dataPreFade = _TrackedData(
destMaterialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == destMaterialElement),
),
);
_expectMaterialPropertiesHaveAdvanced(
smallerMaterial: dataClosed,
biggerMaterial: dataPreFade,
tester: tester,
);
expect(_getOpacity(tester, 'Open'), moreOrLessEquals(0.0));
expect(_getOpacity(tester, 'Closed'), 1.0);
// Jump to the middle of the fade in.
await tester
.pump(const Duration(milliseconds: 30)); // 300ms * 3/10 = 90ms
final _TrackedData dataMidFadeIn = _TrackedData(
destMaterialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == destMaterialElement),
),
);
_expectMaterialPropertiesHaveAdvanced(
smallerMaterial: dataPreFade,
biggerMaterial: dataMidFadeIn,
tester: tester,
);
expect(dataMidFadeIn.material.color, isNot(dataPreFade.material.color));
expect(_getOpacity(tester, 'Open'), lessThan(1.0));
expect(_getOpacity(tester, 'Open'), greaterThan(0.0));
expect(_getOpacity(tester, 'Closed'), 1.0);
// Jump to the end of the fade in at 2/5 of 300ms.
await tester.pump(
const Duration(milliseconds: 30),
); // 300ms * 2/5 = 120ms
final _TrackedData dataPostFadeIn = _TrackedData(
destMaterialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == destMaterialElement),
),
);
_expectMaterialPropertiesHaveAdvanced(
smallerMaterial: dataMidFadeIn,
biggerMaterial: dataPostFadeIn,
tester: tester,
);
expect(_getOpacity(tester, 'Open'), moreOrLessEquals(1.0));
expect(_getOpacity(tester, 'Closed'), 1.0);
// Jump almost to the end of the transition.
await tester.pump(const Duration(milliseconds: 180));
final _TrackedData dataTransitionDone = _TrackedData(
destMaterialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == destMaterialElement),
),
);
_expectMaterialPropertiesHaveAdvanced(
smallerMaterial: dataMidFadeIn,
biggerMaterial: dataTransitionDone,
tester: tester,
);
expect(_getOpacity(tester, 'Open'), 1.0);
expect(dataTransitionDone.material.color, Colors.blue);
expect(dataTransitionDone.material.elevation, 8.0);
expect(dataTransitionDone.radius, 0.0);
expect(dataTransitionDone.rect, const Rect.fromLTRB(0, 0, 800, 600));
await tester.pump(const Duration(milliseconds: 1));
expect(find.text('Closed'), findsNothing); // No longer in the tree.
expect(find.text('Open'), findsOneWidget);
final StatefulElement finalMaterialElement = tester.firstElement(
find.ancestor(
of: find.text('Open'),
matching: find.byType(Material),
),
);
final _TrackedData dataOpen = _TrackedData(
finalMaterialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == finalMaterialElement),
),
);
expect(dataOpen.material.color, dataTransitionDone.material.color);
expect(
dataOpen.material.elevation, dataTransitionDone.material.elevation);
expect(dataOpen.radius, dataTransitionDone.radius);
expect(dataOpen.rect, dataTransitionDone.rect);
},
);
testWidgets(
'Container closes - Fade (by default)',
(WidgetTester tester) async {
const ShapeBorder shape = RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(8.0)),
);
await tester.pumpWidget(_boilerplate(
child: Center(
child: OpenContainer(
closedColor: Colors.green,
openColor: Colors.blue,
closedElevation: 4.0,
openElevation: 8.0,
closedShape: shape,
closedBuilder: (BuildContext context, VoidCallback _) {
return const Text('Closed');
},
openBuilder: (BuildContext context, VoidCallback _) {
return const Text('Open');
},
),
),
));
await tester.tap(find.text('Closed'));
await tester.pumpAndSettle();
// Open container has the expected properties.
expect(find.text('Closed'), findsNothing);
expect(find.text('Open'), findsOneWidget);
final StatefulElement initialMaterialElement = tester.firstElement(
find.ancestor(
of: find.text('Open'),
matching: find.byType(Material),
),
);
final _TrackedData dataOpen = _TrackedData(
initialMaterialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == initialMaterialElement),
),
);
expect(dataOpen.material.color, Colors.blue);
expect(dataOpen.material.elevation, 8.0);
expect(dataOpen.radius, 0.0);
expect(dataOpen.rect, const Rect.fromLTRB(0, 0, 800, 600));
// Close the container.
final NavigatorState navigator = tester.state(find.byType(Navigator));
navigator.pop();
await tester.pump();
expect(find.text('Closed'), findsOneWidget);
expect(find.text('Open'), findsOneWidget);
final StatefulElement materialElement = tester.firstElement(
find.ancestor(
of: find.text('Open'),
matching: find.byType(Material),
),
);
final _TrackedData dataTransitionStart = _TrackedData(
materialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == materialElement),
),
);
expect(dataTransitionStart.material.color, dataOpen.material.color);
expect(
dataTransitionStart.material.elevation, dataOpen.material.elevation);
expect(dataTransitionStart.radius, dataOpen.radius);
expect(dataTransitionStart.rect, dataOpen.rect);
expect(_getOpacity(tester, 'Open'), 1.0);
// Jump to start of fade out: 1/5 of 300.
await tester.pump(const Duration(milliseconds: 60)); // 300 * 1/5 = 60
final _TrackedData dataPreFadeOut = _TrackedData(
materialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == materialElement),
),
);
_expectMaterialPropertiesHaveAdvanced(
smallerMaterial: dataPreFadeOut,
biggerMaterial: dataTransitionStart,
tester: tester,
);
expect(_getOpacity(tester, 'Open'), moreOrLessEquals(1.0));
expect(_getOpacity(tester, 'Closed'), 1.0);
// Jump to the middle of the fade out.
await tester.pump(const Duration(milliseconds: 30)); // 300 * 3/10 = 90
final _TrackedData dataMidpoint = _TrackedData(
materialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == materialElement),
),
);
_expectMaterialPropertiesHaveAdvanced(
smallerMaterial: dataMidpoint,
biggerMaterial: dataPreFadeOut,
tester: tester,
);
expect(dataMidpoint.material.color, isNot(dataPreFadeOut.material.color));
expect(_getOpacity(tester, 'Open'), lessThan(1.0));
expect(_getOpacity(tester, 'Open'), greaterThan(0.0));
expect(_getOpacity(tester, 'Closed'), 1.0);
// Jump to the end of the fade out.
await tester.pump(const Duration(milliseconds: 30)); // 300 * 2/5 = 120
final _TrackedData dataPostFadeOut = _TrackedData(
materialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == materialElement),
),
);
_expectMaterialPropertiesHaveAdvanced(
smallerMaterial: dataPostFadeOut,
biggerMaterial: dataMidpoint,
tester: tester,
);
expect(_getOpacity(tester, 'Open'), moreOrLessEquals(0.0));
expect(_getOpacity(tester, 'Closed'), 1.0);
// Jump almost to the end of the transition.
await tester.pump(const Duration(milliseconds: 180));
final _TrackedData dataTransitionDone = _TrackedData(
materialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == materialElement),
),
);
_expectMaterialPropertiesHaveAdvanced(
smallerMaterial: dataTransitionDone,
biggerMaterial: dataPostFadeOut,
tester: tester,
);
expect(_getOpacity(tester, 'Closed'), 1.0);
expect(_getOpacity(tester, 'Open'), 0.0);
expect(dataTransitionDone.material.color, Colors.green);
expect(dataTransitionDone.material.elevation, 4.0);
expect(dataTransitionDone.radius, 8.0);
await tester.pump(const Duration(milliseconds: 1));
expect(find.text('Open'), findsNothing); // No longer in the tree.
expect(find.text('Closed'), findsOneWidget);
final StatefulElement finalMaterialElement = tester.firstElement(
find.ancestor(
of: find.text('Closed'),
matching: find.byType(Material),
),
);
final _TrackedData dataClosed = _TrackedData(
finalMaterialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == finalMaterialElement),
),
);
expect(dataClosed.material.color, dataTransitionDone.material.color);
expect(
dataClosed.material.elevation,
dataTransitionDone.material.elevation,
);
expect(dataClosed.radius, dataTransitionDone.radius);
expect(dataClosed.rect, dataTransitionDone.rect);
},
);
testWidgets('Container opens - Fade through', (WidgetTester tester) async {
const ShapeBorder shape = RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(8.0)),
);
bool closedBuilderCalled = false;
bool openBuilderCalled = false;
await tester.pumpWidget(_boilerplate(
child: Center(
child: OpenContainer(
closedColor: Colors.green,
openColor: Colors.blue,
middleColor: Colors.red,
closedElevation: 4.0,
openElevation: 8.0,
closedShape: shape,
closedBuilder: (BuildContext context, VoidCallback _) {
closedBuilderCalled = true;
return const Text('Closed');
},
openBuilder: (BuildContext context, VoidCallback _) {
openBuilderCalled = true;
return const Text('Open');
},
transitionType: ContainerTransitionType.fadeThrough,
),
),
));
// Closed container has the expected properties.
final StatefulElement srcMaterialElement = tester.firstElement(
find.ancestor(
of: find.text('Closed'),
matching: find.byType(Material),
),
);
final Material srcMaterial = srcMaterialElement.widget as Material;
expect(srcMaterial.color, Colors.green);
expect(srcMaterial.elevation, 4.0);
expect(srcMaterial.shape, shape);
expect(find.text('Closed'), findsOneWidget);
expect(find.text('Open'), findsNothing);
expect(closedBuilderCalled, isTrue);
expect(openBuilderCalled, isFalse);
final Rect srcMaterialRect = tester.getRect(
find.byElementPredicate((Element e) => e == srcMaterialElement),
);
// Open the container.
await tester.tap(find.text('Closed'));
expect(find.text('Closed'), findsOneWidget);
expect(find.text('Open'), findsNothing);
await tester.pump();
// On the first frame of the animation everything still looks like before.
final StatefulElement destMaterialElement = tester.firstElement(
find.ancestor(
of: find.text('Closed'),
matching: find.byType(Material),
),
);
final Material closedMaterial = destMaterialElement.widget as Material;
expect(closedMaterial.color, Colors.green);
expect(closedMaterial.elevation, 4.0);
expect(closedMaterial.shape, shape);
expect(find.text('Closed'), findsOneWidget);
expect(find.text('Open'), findsOneWidget);
final Rect closedMaterialRect = tester.getRect(
find.byElementPredicate((Element e) => e == destMaterialElement),
);
expect(closedMaterialRect, srcMaterialRect);
expect(_getOpacity(tester, 'Open'), 0.0);
expect(_getOpacity(tester, 'Closed'), 1.0);
final _TrackedData dataClosed = _TrackedData(
closedMaterial,
closedMaterialRect,
);
// The fade-out takes 1/5 of 300ms. Let's jump to the midpoint of that.
await tester.pump(const Duration(milliseconds: 30)); // 300ms * 1/10 = 30ms
final _TrackedData dataMidFadeOut = _TrackedData(
destMaterialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == destMaterialElement),
),
);
_expectMaterialPropertiesHaveAdvanced(
smallerMaterial: dataClosed,
biggerMaterial: dataMidFadeOut,
tester: tester,
);
expect(dataMidFadeOut.material.color, isNot(dataClosed.material.color));
expect(_getOpacity(tester, 'Open'), 0.0);
expect(_getOpacity(tester, 'Closed'), lessThan(1.0));
expect(_getOpacity(tester, 'Closed'), greaterThan(0.0));
// Let's jump to the crossover point at 1/5 of 300ms.
await tester.pump(const Duration(milliseconds: 30)); // 300ms * 1/5 = 60ms
final _TrackedData dataMidpoint = _TrackedData(
destMaterialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == destMaterialElement),
),
);
_expectMaterialPropertiesHaveAdvanced(
smallerMaterial: dataMidFadeOut,
biggerMaterial: dataMidpoint,
tester: tester,
);
expect(dataMidpoint.material.color, Colors.red);
expect(_getOpacity(tester, 'Open'), moreOrLessEquals(0.0));
expect(_getOpacity(tester, 'Closed'), moreOrLessEquals(0.0));
// Let's jump to the middle of the fade-in at 3/5 of 300ms
await tester.pump(const Duration(milliseconds: 120)); // 300ms * 3/5 = 180ms
final _TrackedData dataMidFadeIn = _TrackedData(
destMaterialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == destMaterialElement),
),
);
_expectMaterialPropertiesHaveAdvanced(
smallerMaterial: dataMidpoint,
biggerMaterial: dataMidFadeIn,
tester: tester,
);
expect(dataMidFadeIn.material.color, isNot(dataMidpoint.material.color));
expect(_getOpacity(tester, 'Open'), lessThan(1.0));
expect(_getOpacity(tester, 'Open'), greaterThan(0.0));
expect(_getOpacity(tester, 'Closed'), 0.0);
// Let's jump almost to the end of the transition.
await tester.pump(const Duration(milliseconds: 120));
final _TrackedData dataTransitionDone = _TrackedData(
destMaterialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == destMaterialElement),
),
);
_expectMaterialPropertiesHaveAdvanced(
smallerMaterial: dataMidFadeIn,
biggerMaterial: dataTransitionDone,
tester: tester,
);
expect(
dataTransitionDone.material.color,
isNot(dataMidFadeIn.material.color),
);
expect(_getOpacity(tester, 'Open'), 1.0);
expect(_getOpacity(tester, 'Closed'), 0.0);
expect(dataTransitionDone.material.color, Colors.blue);
expect(dataTransitionDone.material.elevation, 8.0);
expect(dataTransitionDone.radius, 0.0);
expect(dataTransitionDone.rect, const Rect.fromLTRB(0, 0, 800, 600));
await tester.pump(const Duration(milliseconds: 1));
expect(find.text('Closed'), findsNothing); // No longer in the tree.
expect(find.text('Open'), findsOneWidget);
final StatefulElement finalMaterialElement = tester.firstElement(
find.ancestor(
of: find.text('Open'),
matching: find.byType(Material),
),
);
final _TrackedData dataOpen = _TrackedData(
finalMaterialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == finalMaterialElement),
),
);
expect(dataOpen.material.color, dataTransitionDone.material.color);
expect(dataOpen.material.elevation, dataTransitionDone.material.elevation);
expect(dataOpen.radius, dataTransitionDone.radius);
expect(dataOpen.rect, dataTransitionDone.rect);
});
testWidgets('Container closes - Fade through', (WidgetTester tester) async {
const ShapeBorder shape = RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(8.0)),
);
await tester.pumpWidget(_boilerplate(
child: Center(
child: OpenContainer(
closedColor: Colors.green,
openColor: Colors.blue,
middleColor: Colors.red,
closedElevation: 4.0,
openElevation: 8.0,
closedShape: shape,
closedBuilder: (BuildContext context, VoidCallback _) {
return const Text('Closed');
},
openBuilder: (BuildContext context, VoidCallback _) {
return const Text('Open');
},
transitionType: ContainerTransitionType.fadeThrough,
),
),
));
await tester.tap(find.text('Closed'));
await tester.pumpAndSettle();
// Open container has the expected properties.
expect(find.text('Closed'), findsNothing);
expect(find.text('Open'), findsOneWidget);
final StatefulElement initialMaterialElement = tester.firstElement(
find.ancestor(
of: find.text('Open'),
matching: find.byType(Material),
),
);
final _TrackedData dataOpen = _TrackedData(
initialMaterialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == initialMaterialElement),
),
);
expect(dataOpen.material.color, Colors.blue);
expect(dataOpen.material.elevation, 8.0);
expect(dataOpen.radius, 0.0);
expect(dataOpen.rect, const Rect.fromLTRB(0, 0, 800, 600));
// Close the container.
final NavigatorState navigator = tester.state(find.byType(Navigator));
navigator.pop();
await tester.pump();
await tester.pump();
expect(find.text('Closed'), findsOneWidget);
expect(find.text('Open'), findsOneWidget);
final StatefulElement materialElement = tester.firstElement(
find.ancestor(
of: find.text('Open'),
matching: find.byType(Material),
),
);
final _TrackedData dataTransitionStart = _TrackedData(
materialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == materialElement),
),
);
expect(dataTransitionStart.material.color, dataOpen.material.color);
expect(dataTransitionStart.material.elevation, dataOpen.material.elevation);
expect(dataTransitionStart.radius, dataOpen.radius);
expect(dataTransitionStart.rect, dataOpen.rect);
expect(_getOpacity(tester, 'Open'), 1.0);
expect(_getOpacity(tester, 'Closed'), 0.0);
// Jump to mid-point of fade-out: 1/10 of 300ms.
await tester.pump(const Duration(milliseconds: 30)); // 300ms * 1/10 = 30ms
final _TrackedData dataMidFadeOut = _TrackedData(
materialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == materialElement),
),
);
_expectMaterialPropertiesHaveAdvanced(
smallerMaterial: dataMidFadeOut,
biggerMaterial: dataTransitionStart,
tester: tester,
);
expect(
dataMidFadeOut.material.color,
isNot(dataTransitionStart.material.color),
);
expect(_getOpacity(tester, 'Closed'), 0.0);
expect(_getOpacity(tester, 'Open'), lessThan(1.0));
expect(_getOpacity(tester, 'Open'), greaterThan(0.0));
// Let's jump to the crossover point at 1/5 of 300ms.
await tester.pump(const Duration(milliseconds: 30)); // 300ms * 1/5 = 60ms
final _TrackedData dataMidpoint = _TrackedData(
materialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == materialElement),
),
);
_expectMaterialPropertiesHaveAdvanced(
smallerMaterial: dataMidpoint,
biggerMaterial: dataMidFadeOut,
tester: tester,
);
expect(dataMidpoint.material.color, Colors.red);
expect(_getOpacity(tester, 'Open'), moreOrLessEquals(0.0));
expect(_getOpacity(tester, 'Closed'), moreOrLessEquals(0.0));
// Let's jump to the middle of the fade-in at 3/5 of 300ms
await tester.pump(const Duration(milliseconds: 120)); // 300ms * 3/5 = 180ms
final _TrackedData dataMidFadeIn = _TrackedData(
materialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == materialElement),
),
);
_expectMaterialPropertiesHaveAdvanced(
smallerMaterial: dataMidFadeIn,
biggerMaterial: dataMidpoint,
tester: tester,
);
expect(dataMidFadeIn.material.color, isNot(dataMidpoint.material.color));
expect(_getOpacity(tester, 'Closed'), lessThan(1.0));
expect(_getOpacity(tester, 'Closed'), greaterThan(0.0));
expect(_getOpacity(tester, 'Open'), 0.0);
// Let's jump almost to the end of the transition.
await tester.pump(const Duration(milliseconds: 120));
final _TrackedData dataTransitionDone = _TrackedData(
materialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == materialElement),
),
);
_expectMaterialPropertiesHaveAdvanced(
smallerMaterial: dataTransitionDone,
biggerMaterial: dataMidFadeIn,
tester: tester,
);
expect(
dataTransitionDone.material.color,
isNot(dataMidFadeIn.material.color),
);
expect(_getOpacity(tester, 'Closed'), 1.0);
expect(_getOpacity(tester, 'Open'), 0.0);
expect(dataTransitionDone.material.color, Colors.green);
expect(dataTransitionDone.material.elevation, 4.0);
expect(dataTransitionDone.radius, 8.0);
await tester.pump(const Duration(milliseconds: 1));
expect(find.text('Open'), findsNothing); // No longer in the tree.
expect(find.text('Closed'), findsOneWidget);
final StatefulElement finalMaterialElement = tester.firstElement(
find.ancestor(
of: find.text('Closed'),
matching: find.byType(Material),
),
);
final _TrackedData dataClosed = _TrackedData(
finalMaterialElement.widget as Material,
tester.getRect(
find.byElementPredicate((Element e) => e == finalMaterialElement),
),
);
expect(dataClosed.material.color, dataTransitionDone.material.color);
expect(
dataClosed.material.elevation,
dataTransitionDone.material.elevation,
);
expect(dataClosed.radius, dataTransitionDone.radius);
expect(dataClosed.rect, dataTransitionDone.rect);
});
testWidgets('Cannot tap container if tappable=false',
(WidgetTester tester) async {
await tester.pumpWidget(_boilerplate(
child: Center(
child: OpenContainer(
tappable: false,
closedBuilder: (BuildContext context, VoidCallback _) {
return const Text('Closed');
},
openBuilder: (BuildContext context, VoidCallback _) {
return const Text('Open');
},
),
),
));
expect(find.text('Open'), findsNothing);
expect(find.text('Closed'), findsOneWidget);
await tester.tap(find.text('Closed'));
await tester.pumpAndSettle();
expect(find.text('Open'), findsNothing);
expect(find.text('Closed'), findsOneWidget);
});
testWidgets('Action callbacks work', (WidgetTester tester) async {
late VoidCallback open, close;
await tester.pumpWidget(_boilerplate(
child: Center(
child: OpenContainer(
tappable: false,
closedBuilder: (BuildContext context, VoidCallback action) {
open = action;
return const Text('Closed');
},
openBuilder: (BuildContext context, VoidCallback action) {
close = action;
return const Text('Open');
},
),
),
));
expect(find.text('Open'), findsNothing);
expect(find.text('Closed'), findsOneWidget);
expect(open, isNotNull);
open();
await tester.pumpAndSettle();
expect(find.text('Open'), findsOneWidget);
expect(find.text('Closed'), findsNothing);
expect(close, isNotNull);
close();
await tester.pumpAndSettle();
expect(find.text('Open'), findsNothing);
expect(find.text('Closed'), findsOneWidget);
});
testWidgets('open widget keeps state', (WidgetTester tester) async {
await tester.pumpWidget(_boilerplate(
child: Center(
child: OpenContainer(
closedBuilder: (BuildContext context, VoidCallback action) {
return const Text('Closed');
},
openBuilder: (BuildContext context, VoidCallback action) {
return const DummyStatefulWidget();
},
),
),
));
await tester.tap(find.text('Closed'));
await tester.pump(const Duration(milliseconds: 200));
final State stateOpening = tester.state(find.byType(DummyStatefulWidget));
expect(stateOpening, isNotNull);
await tester.pumpAndSettle();
expect(find.text('Closed'), findsNothing);
final State stateOpen = tester.state(find.byType(DummyStatefulWidget));
expect(stateOpen, isNotNull);
expect(stateOpen, same(stateOpening));
final NavigatorState navigator = tester.state(find.byType(Navigator));
navigator.pop();
await tester.pump(const Duration(milliseconds: 200));
expect(find.text('Closed'), findsOneWidget);
final State stateClosing = tester.state(find.byType(DummyStatefulWidget));
expect(stateClosing, isNotNull);
expect(stateClosing, same(stateOpen));
});
testWidgets('closed widget keeps state', (WidgetTester tester) async {
late VoidCallback open;
await tester.pumpWidget(_boilerplate(
child: Center(
child: OpenContainer(
closedBuilder: (BuildContext context, VoidCallback action) {
open = action;
return const DummyStatefulWidget();
},
openBuilder: (BuildContext context, VoidCallback action) {
return const Text('Open');
},
),
),
));
final State stateClosed = tester.state(find.byType(DummyStatefulWidget));
expect(stateClosed, isNotNull);
open();
await tester.pump(const Duration(milliseconds: 200));
expect(find.text('Open'), findsOneWidget);
final State stateOpening = tester.state(find.byType(DummyStatefulWidget));
expect(stateOpening, same(stateClosed));
await tester.pumpAndSettle();
expect(find.byType(DummyStatefulWidget), findsNothing);
expect(find.text('Open'), findsOneWidget);
final State stateOpen = tester.state(find.byType(
DummyStatefulWidget,
skipOffstage: false,
));
expect(stateOpen, same(stateOpening));
final NavigatorState navigator = tester.state(find.byType(Navigator));
navigator.pop();
await tester.pump(const Duration(milliseconds: 200));
expect(find.text('Open'), findsOneWidget);
final State stateClosing = tester.state(find.byType(DummyStatefulWidget));
expect(stateClosing, same(stateOpen));
await tester.pumpAndSettle();
expect(find.text('Open'), findsNothing);
final State stateClosedAgain =
tester.state(find.byType(DummyStatefulWidget));
expect(stateClosedAgain, same(stateClosing));
});
testWidgets('closes to the right location when src position has changed',
(WidgetTester tester) async {
final Widget openContainer = OpenContainer(
closedBuilder: (BuildContext context, VoidCallback action) {
return const SizedBox(
height: 100,
width: 100,
child: Text('Closed'),
);
},
openBuilder: (BuildContext context, VoidCallback action) {
return GestureDetector(
onTap: action,
child: const Text('Open'),
);
},
);
await tester.pumpWidget(_boilerplate(
child: Align(
alignment: Alignment.topLeft,
child: openContainer,
),
));
final Rect originTextRect = tester.getRect(find.text('Closed'));
expect(originTextRect.topLeft, Offset.zero);
await tester.tap(find.text('Closed'));
await tester.pumpAndSettle();
expect(find.text('Open'), findsOneWidget);
expect(find.text('Closed'), findsNothing);
await tester.pumpWidget(_boilerplate(
child: Align(
alignment: Alignment.bottomLeft,
child: openContainer,
),
));
expect(find.text('Open'), findsOneWidget);
expect(find.text('Closed'), findsNothing);
await tester.tap(find.text('Open'));
await tester.pump(); // Need one frame to measure things in the old route.
await tester.pump(const Duration(milliseconds: 300));
expect(find.text('Open'), findsOneWidget);
expect(find.text('Closed'), findsOneWidget);
final Rect transitionEndTextRect = tester.getRect(find.text('Open'));
expect(transitionEndTextRect.topLeft, const Offset(0.0, 600.0 - 100.0));
await tester.pump(const Duration(milliseconds: 1));
expect(find.text('Open'), findsNothing);
expect(find.text('Closed'), findsOneWidget);
final Rect finalTextRect = tester.getRect(find.text('Closed'));
expect(finalTextRect.topLeft, transitionEndTextRect.topLeft);
});
testWidgets('src changes size while open', (WidgetTester tester) async {
final Widget openContainer = _boilerplate(
child: Center(
child: OpenContainer(
closedBuilder: (BuildContext context, VoidCallback action) {
return const _SizableContainer(
initialSize: 100,
child: Text('Closed'),
);
},
openBuilder: (BuildContext context, VoidCallback action) {
return GestureDetector(
onTap: action,
child: const Text('Open'),
);
},
),
),
);
await tester.pumpWidget(openContainer);
final Size orignalClosedRect = tester.getSize(find
.ancestor(
of: find.text('Closed'),
matching: find.byType(Material),
)
.first);
expect(orignalClosedRect, const Size(100, 100));
await tester.tap(find.text('Closed'));
await tester.pumpAndSettle();
expect(find.text('Open'), findsOneWidget);
expect(find.text('Closed'), findsNothing);
final _SizableContainerState containerState = tester.state(find.byType(
_SizableContainer,
skipOffstage: false,
));
containerState.size = 200;
expect(find.text('Open'), findsOneWidget);
expect(find.text('Closed'), findsNothing);
await tester.tap(find.text('Open'));
await tester.pump(); // Need one frame to measure things in the old route.
await tester.pump(const Duration(milliseconds: 300));
expect(find.text('Open'), findsOneWidget);
expect(find.text('Closed'), findsOneWidget);
final Size transitionEndSize = tester.getSize(find
.ancestor(
of: find.text('Open'),
matching: find.byType(Material),
)
.first);
expect(transitionEndSize, const Size(200, 200));
await tester.pump(const Duration(milliseconds: 1));
expect(find.text('Open'), findsNothing);
expect(find.text('Closed'), findsOneWidget);
final Size finalSize = tester.getSize(find
.ancestor(
of: find.text('Closed'),
matching: find.byType(Material),
)
.first);
expect(finalSize, const Size(200, 200));
});
testWidgets('transition is interrupted and should not jump',
(WidgetTester tester) async {
await tester.pumpWidget(_boilerplate(
child: Center(
child: OpenContainer(
closedBuilder: (BuildContext context, VoidCallback action) {
return const Text('Closed');
},
openBuilder: (BuildContext context, VoidCallback action) {
return const Text('Open');
},
),
),
));
await tester.tap(find.text('Closed'));
await tester.pump();
await tester.pump(const Duration(milliseconds: 150));
expect(find.text('Open'), findsOneWidget);
expect(find.text('Closed'), findsOneWidget);
final Material openingMaterial = tester.firstWidget(find.ancestor(
of: find.text('Closed'),
matching: find.byType(Material),
));
final Rect openingRect = tester.getRect(
find.byWidgetPredicate((Widget w) => w == openingMaterial),
);
// Close the container while it is half way to open.
final NavigatorState navigator = tester.state(find.byType(Navigator));
navigator.pop();
await tester.pump();
final Material closingMaterial = tester.firstWidget(find.ancestor(
of: find.text('Open'),
matching: find.byType(Material),
));
final Rect closingRect = tester.getRect(
find.byWidgetPredicate((Widget w) => w == closingMaterial),
);
expect(closingMaterial.elevation, openingMaterial.elevation);
expect(closingMaterial.color, openingMaterial.color);
expect(closingMaterial.shape, openingMaterial.shape);
expect(closingRect, openingRect);
});
testWidgets('navigator is not full size', (WidgetTester tester) async {
await tester.pumpWidget(Center(
child: SizedBox(
width: 300,
height: 400,
child: _boilerplate(
child: Center(
child: OpenContainer(
closedBuilder: (BuildContext context, VoidCallback action) {
return const Text('Closed');
},
openBuilder: (BuildContext context, VoidCallback action) {
return const Text('Open');
},
),
),
),
),
));
const Rect fullNavigator = Rect.fromLTWH(250, 100, 300, 400);
expect(tester.getRect(find.byType(Navigator)), fullNavigator);
final Rect materialRectClosed = tester.getRect(find
.ancestor(
of: find.text('Closed'),
matching: find.byType(Material),
)
.first);
await tester.tap(find.text('Closed'));
await tester.pump();
expect(find.text('Open'), findsOneWidget);
expect(find.text('Closed'), findsOneWidget);
final Rect materialRectTransitionStart = tester.getRect(find.ancestor(
of: find.text('Closed'),
matching: find.byType(Material),
));
expect(materialRectTransitionStart, materialRectClosed);
await tester.pump(const Duration(milliseconds: 300));
expect(find.text('Open'), findsOneWidget);
expect(find.text('Closed'), findsOneWidget);
final Rect materialRectTransitionEnd = tester.getRect(find.ancestor(
of: find.text('Open'),
matching: find.byType(Material),
));
expect(materialRectTransitionEnd, fullNavigator);
await tester.pumpAndSettle();
expect(find.text('Open'), findsOneWidget);
expect(find.text('Closed'), findsNothing);
final Rect materialRectOpen = tester.getRect(find.ancestor(
of: find.text('Open'),
matching: find.byType(Material),
));
expect(materialRectOpen, fullNavigator);
});
testWidgets('does not crash when disposed right after pop',
(WidgetTester tester) async {
await tester.pumpWidget(Center(
child: SizedBox(
width: 300,
height: 400,
child: _boilerplate(
child: Center(
child: OpenContainer(
closedBuilder: (BuildContext context, VoidCallback action) {
return const Text('Closed');
},
openBuilder: (BuildContext context, VoidCallback action) {
return const Text('Open');
},
),
),
),
),
));
await tester.tap(find.text('Closed'));
await tester.pumpAndSettle();
final NavigatorState navigator = tester.state(find.byType(Navigator));
navigator.pop();
await tester.pumpWidget(const Placeholder());
expect(tester.takeException(), isNull);
await tester.pumpAndSettle();
expect(tester.takeException(), isNull);
});
testWidgets('can specify a duration', (WidgetTester tester) async {
await tester.pumpWidget(Center(
child: SizedBox(
width: 300,
height: 400,
child: _boilerplate(
child: Center(
child: OpenContainer(
transitionDuration: const Duration(seconds: 2),
closedBuilder: (BuildContext context, VoidCallback action) {
return const Text('Closed');
},
openBuilder: (BuildContext context, VoidCallback action) {
return const Text('Open');
},
),
),
),
),
));
expect(find.text('Open'), findsNothing);
expect(find.text('Closed'), findsOneWidget);
await tester.tap(find.text('Closed'));
await tester.pump();
// Jump to the end of the transition.
await tester.pump(const Duration(seconds: 2));
expect(find.text('Open'), findsOneWidget); // faded in
expect(find.text('Closed'), findsOneWidget); // faded out
await tester.pump(const Duration(milliseconds: 1));
expect(find.text('Open'), findsOneWidget);
expect(find.text('Closed'), findsNothing);
final NavigatorState navigator = tester.state(find.byType(Navigator));
navigator.pop();
await tester.pump();
// Jump to the end of the transition.
await tester.pump(const Duration(seconds: 2));
expect(find.text('Open'), findsOneWidget); // faded out
expect(find.text('Closed'), findsOneWidget); // faded in
await tester.pump(const Duration(milliseconds: 1));
expect(find.text('Open'), findsNothing);
expect(find.text('Closed'), findsOneWidget);
});
testWidgets('can specify an open shape', (WidgetTester tester) async {
await tester.pumpWidget(Center(
child: SizedBox(
width: 300,
height: 400,
child: _boilerplate(
child: Center(
child: OpenContainer(
closedShape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10),
),
openShape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(40),
),
closedBuilder: (BuildContext context, VoidCallback action) {
return const Text('Closed');
},
openBuilder: (BuildContext context, VoidCallback action) {
return const Text('Open');
},
),
),
),
),
));
expect(find.text('Open'), findsNothing);
expect(find.text('Closed'), findsOneWidget);
final double closedRadius = _getRadius(tester.firstWidget(find.ancestor(
of: find.text('Closed'),
matching: find.byType(Material),
)));
expect(closedRadius, 10.0);
await tester.tap(find.text('Closed'));
await tester.pump();
expect(find.text('Open'), findsOneWidget);
expect(find.text('Closed'), findsOneWidget);
final double openingRadius = _getRadius(tester.firstWidget(find.ancestor(
of: find.text('Open'),
matching: find.byType(Material),
)));
expect(openingRadius, 10.0);
await tester.pump(const Duration(milliseconds: 150));
expect(find.text('Open'), findsOneWidget);
expect(find.text('Closed'), findsOneWidget);
final double halfwayRadius = _getRadius(tester.firstWidget(find.ancestor(
of: find.text('Open'),
matching: find.byType(Material),
)));
expect(halfwayRadius, greaterThan(10.0));
expect(halfwayRadius, lessThan(40.0));
await tester.pump(const Duration(milliseconds: 150));
expect(find.text('Open'), findsOneWidget);
expect(find.text('Closed'), findsOneWidget);
final double openRadius = _getRadius(tester.firstWidget(find.ancestor(
of: find.text('Open'),
matching: find.byType(Material),
)));
expect(openRadius, 40.0);
await tester.pump(const Duration(milliseconds: 1));
expect(find.text('Closed'), findsNothing);
expect(find.text('Open'), findsOneWidget);
final double finalRadius = _getRadius(tester.firstWidget(find.ancestor(
of: find.text('Open'),
matching: find.byType(Material),
)));
expect(finalRadius, 40.0);
});
testWidgets('Scrim', (WidgetTester tester) async {
const ShapeBorder shape = RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(8.0)),
);
await tester.pumpWidget(_boilerplate(
child: Center(
child: OpenContainer(
closedColor: Colors.green,
openColor: Colors.blue,
closedElevation: 4.0,
openElevation: 8.0,
closedShape: shape,
closedBuilder: (BuildContext context, VoidCallback _) {
return const Text('Closed');
},
openBuilder: (BuildContext context, VoidCallback _) {
return const Text('Open');
},
),
),
));
expect(find.text('Closed'), findsOneWidget);
expect(find.text('Open'), findsNothing);
await tester.tap(find.text('Closed'));
await tester.pump();
expect(_getScrimColor(tester), Colors.transparent);
await tester.pump(const Duration(milliseconds: 50));
final Color halfwayFadeInColor = _getScrimColor(tester);
expect(halfwayFadeInColor, isNot(Colors.transparent));
expect(halfwayFadeInColor, isNot(Colors.black54));
// Scrim is done fading in early.
await tester.pump(const Duration(milliseconds: 50));
expect(_getScrimColor(tester), Colors.black54);
await tester.pump(const Duration(milliseconds: 200));
expect(_getScrimColor(tester), Colors.black54);
await tester.pumpAndSettle();
// Close the container
final NavigatorState navigator = tester.state(find.byType(Navigator));
navigator.pop();
await tester.pump();
expect(_getScrimColor(tester), Colors.black54);
// Scrim takes longer to fade out (vs. fade in).
await tester.pump(const Duration(milliseconds: 200));
final Color halfwayFadeOutColor = _getScrimColor(tester);
expect(halfwayFadeOutColor, isNot(Colors.transparent));
expect(halfwayFadeOutColor, isNot(Colors.black54));
await tester.pump(const Duration(milliseconds: 100));
expect(_getScrimColor(tester), Colors.transparent);
});
testWidgets(
'Container partly offscreen can be opened without crash - vertical',
(WidgetTester tester) async {
final ScrollController controller =
ScrollController(initialScrollOffset: 50);
await tester.pumpWidget(Center(
child: SizedBox(
height: 200,
width: 200,
child: _boilerplate(
child: ListView.builder(
cacheExtent: 0,
controller: controller,
itemBuilder: (BuildContext context, int index) {
return OpenContainer(
closedBuilder: (BuildContext context, VoidCallback _) {
return SizedBox(
height: 100,
width: 100,
child: Text('Closed $index'),
);
},
openBuilder: (BuildContext context, VoidCallback _) {
return Text('Open $index');
},
);
},
),
),
),
));
void expectClosedState() {
expect(find.text('Closed 0'), findsOneWidget);
expect(find.text('Closed 1'), findsOneWidget);
expect(find.text('Closed 2'), findsOneWidget);
expect(find.text('Closed 3'), findsNothing);
expect(find.text('Open 0'), findsNothing);
expect(find.text('Open 1'), findsNothing);
expect(find.text('Open 2'), findsNothing);
expect(find.text('Open 3'), findsNothing);
}
expectClosedState();
// Open container that's partly visible at top.
await tester.tapAt(
tester.getBottomRight(find.text('Closed 0')) - const Offset(20, 20),
);
await tester.pump();
await tester.pumpAndSettle();
expect(find.text('Closed 0'), findsNothing);
expect(find.text('Open 0'), findsOneWidget);
final NavigatorState navigator = tester.state(find.byType(Navigator));
navigator.pop();
await tester.pump();
await tester.pumpAndSettle();
expectClosedState();
// Open container that's partly visible at bottom.
await tester.tapAt(
tester.getTopLeft(find.text('Closed 2')) + const Offset(20, 20),
);
await tester.pump();
await tester.pumpAndSettle();
expect(find.text('Closed 2'), findsNothing);
expect(find.text('Open 2'), findsOneWidget);
});
testWidgets(
'Container partly offscreen can be opened without crash - horizontal',
(WidgetTester tester) async {
final ScrollController controller =
ScrollController(initialScrollOffset: 50);
await tester.pumpWidget(Center(
child: SizedBox(
height: 200,
width: 200,
child: _boilerplate(
child: ListView.builder(
scrollDirection: Axis.horizontal,
cacheExtent: 0,
controller: controller,
itemBuilder: (BuildContext context, int index) {
return OpenContainer(
closedBuilder: (BuildContext context, VoidCallback _) {
return SizedBox(
height: 100,
width: 100,
child: Text('Closed $index'),
);
},
openBuilder: (BuildContext context, VoidCallback _) {
return Text('Open $index');
},
);
},
),
),
),
));
void expectClosedState() {
expect(find.text('Closed 0'), findsOneWidget);
expect(find.text('Closed 1'), findsOneWidget);
expect(find.text('Closed 2'), findsOneWidget);
expect(find.text('Closed 3'), findsNothing);
expect(find.text('Open 0'), findsNothing);
expect(find.text('Open 1'), findsNothing);
expect(find.text('Open 2'), findsNothing);
expect(find.text('Open 3'), findsNothing);
}
expectClosedState();
// Open container that's partly visible at left edge.
await tester.tapAt(
tester.getBottomRight(find.text('Closed 0')) - const Offset(20, 20),
);
await tester.pump();
await tester.pumpAndSettle();
expect(find.text('Closed 0'), findsNothing);
expect(find.text('Open 0'), findsOneWidget);
final NavigatorState navigator = tester.state(find.byType(Navigator));
navigator.pop();
await tester.pump();
await tester.pumpAndSettle();
expectClosedState();
// Open container that's partly visible at right edge.
await tester.tapAt(
tester.getTopLeft(find.text('Closed 2')) + const Offset(20, 20),
);
await tester.pump();
await tester.pumpAndSettle();
expect(find.text('Closed 2'), findsNothing);
expect(find.text('Open 2'), findsOneWidget);
});
testWidgets(
'Container can be dismissed after container widget itself is removed without crash',
(WidgetTester tester) async {
await tester.pumpWidget(_boilerplate(child: _RemoveOpenContainerExample()));
expect(find.text('Closed'), findsOneWidget);
expect(find.text('Closed', skipOffstage: false), findsOneWidget);
expect(find.text('Open'), findsNothing);
await tester.tap(find.text('Open the container'));
await tester.pumpAndSettle();
expect(find.text('Closed'), findsNothing);
expect(find.text('Closed', skipOffstage: false), findsOneWidget);
expect(find.text('Open'), findsOneWidget);
await tester.tap(find.text('Remove the container'));
await tester.pump();
expect(find.text('Closed'), findsNothing);
expect(find.text('Closed', skipOffstage: false), findsNothing);
expect(find.text('Open'), findsOneWidget);
await tester.tap(find.text('Close the container'));
await tester.pumpAndSettle();
expect(find.text('Closed'), findsNothing);
expect(find.text('Closed', skipOffstage: false), findsNothing);
expect(find.text('Open'), findsNothing);
expect(find.text('Container has been removed'), findsOneWidget);
});
testWidgets('onClosed callback is called when container has closed',
(WidgetTester tester) async {
bool hasClosed = false;
final Widget openContainer = OpenContainer(
onClosed: (dynamic _) {
hasClosed = true;
},
closedBuilder: (BuildContext context, VoidCallback action) {
return GestureDetector(
onTap: action,
child: const Text('Closed'),
);
},
openBuilder: (BuildContext context, VoidCallback action) {
return GestureDetector(
onTap: action,
child: const Text('Open'),
);
},
);
await tester.pumpWidget(
_boilerplate(child: openContainer),
);
expect(find.text('Open'), findsNothing);
expect(find.text('Closed'), findsOneWidget);
expect(hasClosed, isFalse);
await tester.tap(find.text('Closed'));
await tester.pumpAndSettle();
expect(find.text('Open'), findsOneWidget);
expect(find.text('Closed'), findsNothing);
await tester.tap(find.text('Open'));
await tester.pumpAndSettle();
expect(find.text('Open'), findsNothing);
expect(find.text('Closed'), findsOneWidget);
expect(hasClosed, isTrue);
});
testWidgets(
'onClosed callback receives popped value when container has closed',
(WidgetTester tester) async {
bool? value = false;
final Widget openContainer = OpenContainer<bool>(
onClosed: (bool? poppedValue) {
value = poppedValue;
},
closedBuilder: (BuildContext context, VoidCallback action) {
return GestureDetector(
onTap: action,
child: const Text('Closed'),
);
},
openBuilder:
(BuildContext context, CloseContainerActionCallback<bool> action) {
return GestureDetector(
onTap: () => action(returnValue: true),
child: const Text('Open'),
);
},
);
await tester.pumpWidget(
_boilerplate(child: openContainer),
);
expect(find.text('Open'), findsNothing);
expect(find.text('Closed'), findsOneWidget);
expect(value, isFalse);
await tester.tap(find.text('Closed'));
await tester.pumpAndSettle();
expect(find.text('Open'), findsOneWidget);
expect(find.text('Closed'), findsNothing);
await tester.tap(find.text('Open'));
await tester.pumpAndSettle();
expect(find.text('Open'), findsNothing);
expect(find.text('Closed'), findsOneWidget);
expect(value, isTrue);
});
testWidgets('closedBuilder has anti-alias clip by default',
(WidgetTester tester) async {
final GlobalKey closedBuilderKey = GlobalKey();
final Widget openContainer = OpenContainer(
closedBuilder: (BuildContext context, VoidCallback action) {
return Text('Close', key: closedBuilderKey);
},
openBuilder:
(BuildContext context, CloseContainerActionCallback<bool> action) {
return const Text('Open');
},
);
await tester.pumpWidget(
_boilerplate(child: openContainer),
);
final Finder closedBuilderMaterial = find
.ancestor(
of: find.byKey(closedBuilderKey),
matching: find.byType(Material),
)
.first;
final Material material = tester.widget<Material>(closedBuilderMaterial);
expect(material.clipBehavior, Clip.antiAlias);
});
testWidgets('closedBuilder has no clip', (WidgetTester tester) async {
final GlobalKey closedBuilderKey = GlobalKey();
final Widget openContainer = OpenContainer(
closedBuilder: (BuildContext context, VoidCallback action) {
return Text('Close', key: closedBuilderKey);
},
openBuilder:
(BuildContext context, CloseContainerActionCallback<bool> action) {
return const Text('Open');
},
clipBehavior: Clip.none,
);
await tester.pumpWidget(
_boilerplate(child: openContainer),
);
final Finder closedBuilderMaterial = find
.ancestor(
of: find.byKey(closedBuilderKey),
matching: find.byType(Material),
)
.first;
final Material material = tester.widget<Material>(closedBuilderMaterial);
expect(material.clipBehavior, Clip.none);
});
Widget createRootNavigatorTest({
required Key appKey,
required Key nestedNavigatorKey,
required bool useRootNavigator,
}) {
return Center(
child: SizedBox(
width: 100,
height: 100,
child: MaterialApp(
key: appKey,
// a nested navigator
home: Center(
child: SizedBox(
width: 50,
height: 50,
child: Navigator(
key: nestedNavigatorKey,
onGenerateRoute: (RouteSettings route) {
return MaterialPageRoute<dynamic>(
settings: route,
builder: (BuildContext context) {
return OpenContainer(
useRootNavigator: useRootNavigator,
closedBuilder: (BuildContext context, _) {
return const Text('Closed');
},
openBuilder: (BuildContext context, _) {
return const Text('Opened');
},
);
},
);
},
),
),
),
),
),
);
}
testWidgets(
'Verify that "useRootNavigator: false" uses the correct navigator',
(WidgetTester tester) async {
const Key appKey = Key('App');
const Key nestedNavigatorKey = Key('Nested Navigator');
await tester.pumpWidget(createRootNavigatorTest(
appKey: appKey,
nestedNavigatorKey: nestedNavigatorKey,
useRootNavigator: false));
await tester.tap(find.text('Closed'));
await tester.pumpAndSettle();
expect(
find.descendant(of: find.byKey(appKey), matching: find.text('Opened')),
findsOneWidget);
expect(
find.descendant(
of: find.byKey(nestedNavigatorKey), matching: find.text('Opened')),
findsOneWidget);
});
testWidgets('Verify that "useRootNavigator: true" uses the correct navigator',
(WidgetTester tester) async {
const Key appKey = Key('App');
const Key nestedNavigatorKey = Key('Nested Navigator');
await tester.pumpWidget(createRootNavigatorTest(
appKey: appKey,
nestedNavigatorKey: nestedNavigatorKey,
useRootNavigator: true));
await tester.tap(find.text('Closed'));
await tester.pumpAndSettle();
expect(
find.descendant(of: find.byKey(appKey), matching: find.text('Opened')),
findsOneWidget);
expect(
find.descendant(
of: find.byKey(nestedNavigatorKey), matching: find.text('Opened')),
findsNothing);
});
testWidgets('Verify correct opened size when "useRootNavigator: false"',
(WidgetTester tester) async {
const Key appKey = Key('App');
const Key nestedNavigatorKey = Key('Nested Navigator');
await tester.pumpWidget(createRootNavigatorTest(
appKey: appKey,
nestedNavigatorKey: nestedNavigatorKey,
useRootNavigator: false));
await tester.tap(find.text('Closed'));
await tester.pumpAndSettle();
expect(tester.getSize(find.text('Opened')),
equals(tester.getSize(find.byKey(nestedNavigatorKey))));
});
testWidgets('Verify correct opened size when "useRootNavigator: true"',
(WidgetTester tester) async {
const Key appKey = Key('App');
const Key nestedNavigatorKey = Key('Nested Navigator');
await tester.pumpWidget(createRootNavigatorTest(
appKey: appKey,
nestedNavigatorKey: nestedNavigatorKey,
useRootNavigator: true));
await tester.tap(find.text('Closed'));
await tester.pumpAndSettle();
expect(tester.getSize(find.text('Opened')),
equals(tester.getSize(find.byKey(appKey))));
});
testWidgets(
'Verify routeSettings passed to Navigator',
(WidgetTester tester) async {
const RouteSettings routeSettings = RouteSettings(
name: 'route-name',
arguments: 'arguments',
);
final Widget openContainer = OpenContainer(
routeSettings: routeSettings,
closedBuilder: (BuildContext context, VoidCallback action) {
return GestureDetector(
onTap: action,
child: const Text('Closed'),
);
},
openBuilder: (BuildContext context, VoidCallback action) {
return GestureDetector(
onTap: action,
child: const Text('Open'),
);
},
);
await tester.pumpWidget(_boilerplate(child: openContainer));
// Open the container
await tester.tap(find.text('Closed'));
await tester.pumpAndSettle();
// Expect the last route pushed to the navigator to contain RouteSettings
// equal to the RouteSettings passed to the OpenContainer
final ModalRoute<dynamic> modalRoute = ModalRoute.of(
tester.element(find.text('Open')),
)!;
expect(modalRoute.settings, routeSettings);
},
);
}
Color _getScrimColor(WidgetTester tester) {
return tester
.widget<ColoredBox>(
find.descendant(
of: find.byType(Container),
matching: find.byType(ColoredBox),
),
)
.color;
}
void _expectMaterialPropertiesHaveAdvanced({
required _TrackedData biggerMaterial,
required _TrackedData smallerMaterial,
required WidgetTester tester,
}) {
expect(
biggerMaterial.material.elevation,
greaterThan(smallerMaterial.material.elevation),
);
expect(biggerMaterial.radius, lessThan(smallerMaterial.radius));
expect(biggerMaterial.rect.height, greaterThan(smallerMaterial.rect.height));
expect(biggerMaterial.rect.width, greaterThan(smallerMaterial.rect.width));
expect(biggerMaterial.rect.top, lessThan(smallerMaterial.rect.top));
expect(biggerMaterial.rect.left, lessThan(smallerMaterial.rect.left));
}
double _getOpacity(WidgetTester tester, String label) {
final FadeTransition widget = tester.firstWidget(find.ancestor(
of: find.text(label),
matching: find.byType(FadeTransition),
));
// Verify that the correct fade transition is retrieved (i.e. not something from a page transition).
assert(widget.child is Builder && widget.child?.key is GlobalKey, '$widget');
return widget.opacity.value;
}
class _TrackedData {
_TrackedData(this.material, this.rect);
final Material material;
final Rect rect;
double get radius => _getRadius(material);
}
double _getRadius(Material material) {
final RoundedRectangleBorder? shape =
material.shape as RoundedRectangleBorder?;
if (shape == null) {
return 0.0;
}
final BorderRadius radius = shape.borderRadius as BorderRadius;
return radius.topRight.x;
}
Widget _boilerplate({required Widget child}) {
return MaterialApp(
home: Scaffold(
body: child,
),
);
}
class _SizableContainer extends StatefulWidget {
const _SizableContainer({required this.initialSize, required this.child});
final double initialSize;
final Widget child;
@override
State<_SizableContainer> createState() => _SizableContainerState();
}
class _SizableContainerState extends State<_SizableContainer> {
@override
void initState() {
super.initState();
_size = widget.initialSize;
}
double get size => _size;
late double _size;
set size(double value) {
if (value == _size) {
return;
}
setState(() {
_size = value;
});
}
@override
Widget build(BuildContext context) {
return SizedBox(
height: size,
width: size,
child: widget.child,
);
}
}
class _RemoveOpenContainerExample extends StatefulWidget {
@override
__RemoveOpenContainerExampleState createState() =>
__RemoveOpenContainerExampleState();
}
class __RemoveOpenContainerExampleState
extends State<_RemoveOpenContainerExample> {
bool removeOpenContainerWidget = false;
@override
Widget build(BuildContext context) {
return removeOpenContainerWidget
? const Text('Container has been removed')
: OpenContainer(
closedBuilder: (BuildContext context, VoidCallback action) =>
Column(
children: <Widget>[
const Text('Closed'),
ElevatedButton(
onPressed: action,
child: const Text('Open the container'),
),
],
),
openBuilder: (BuildContext context, VoidCallback action) => Column(
children: <Widget>[
const Text('Open'),
ElevatedButton(
onPressed: action,
child: const Text('Close the container'),
),
ElevatedButton(
onPressed: () {
setState(() {
removeOpenContainerWidget = true;
});
},
child: const Text('Remove the container')),
],
),
);
}
}
class DummyStatefulWidget extends StatefulWidget {
const DummyStatefulWidget({super.key});
@override
State<StatefulWidget> createState() => DummyState();
}
class DummyState extends State<DummyStatefulWidget> {
@override
Widget build(BuildContext context) => const SizedBox.expand();
}
| packages/packages/animations/test/open_container_test.dart/0 | {
"file_path": "packages/packages/animations/test/open_container_test.dart",
"repo_id": "packages",
"token_count": 26512
} | 937 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.camerax;
import android.content.Context;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.annotation.VisibleForTesting;
import androidx.camera.core.ImageAnalysis;
import androidx.camera.core.resolutionselector.ResolutionSelector;
import androidx.core.content.ContextCompat;
import io.flutter.plugin.common.BinaryMessenger;
import io.flutter.plugins.camerax.GeneratedCameraXLibrary.ImageAnalysisHostApi;
import java.util.Objects;
public class ImageAnalysisHostApiImpl implements ImageAnalysisHostApi {
private InstanceManager instanceManager;
private BinaryMessenger binaryMessenger;
@Nullable private Context context;
@VisibleForTesting @NonNull public CameraXProxy cameraXProxy = new CameraXProxy();
public ImageAnalysisHostApiImpl(
@NonNull BinaryMessenger binaryMessenger,
@NonNull InstanceManager instanceManager,
@NonNull Context context) {
this.binaryMessenger = binaryMessenger;
this.instanceManager = instanceManager;
this.context = context;
}
/**
* Sets the context that will be used to run an {@link ImageAnalysis.Analyzer} on the main thread.
*/
public void setContext(@NonNull Context context) {
this.context = context;
}
/** Creates an {@link ImageAnalysis} instance with the target resolution if specified. */
@Override
public void create(
@NonNull Long identifier, @Nullable Long rotation, @Nullable Long resolutionSelectorId) {
ImageAnalysis.Builder imageAnalysisBuilder = cameraXProxy.createImageAnalysisBuilder();
if (rotation != null) {
imageAnalysisBuilder.setTargetRotation(rotation.intValue());
}
if (resolutionSelectorId != null) {
ResolutionSelector resolutionSelector =
Objects.requireNonNull(instanceManager.getInstance(resolutionSelectorId));
imageAnalysisBuilder.setResolutionSelector(resolutionSelector);
}
ImageAnalysis imageAnalysis = imageAnalysisBuilder.build();
instanceManager.addDartCreatedInstance(imageAnalysis, identifier);
}
/**
* Sets {@link ImageAnalysis.Analyzer} instance with specified {@code analyzerIdentifier} on the
* {@link ImageAnalysis} instance with the specified {@code identifier} to receive and analyze
* images.
*/
@Override
public void setAnalyzer(@NonNull Long identifier, @NonNull Long analyzerIdentifier) {
if (context == null) {
throw new IllegalStateException("Context must be set to set an Analyzer.");
}
getImageAnalysisInstance(identifier)
.setAnalyzer(
ContextCompat.getMainExecutor(context),
Objects.requireNonNull(instanceManager.getInstance(analyzerIdentifier)));
}
/** Clears any analyzer previously set on the specified {@link ImageAnalysis} instance. */
@Override
public void clearAnalyzer(@NonNull Long identifier) {
ImageAnalysis imageAnalysis =
(ImageAnalysis) Objects.requireNonNull(instanceManager.getInstance(identifier));
imageAnalysis.clearAnalyzer();
}
/** Dynamically sets the target rotation of the {@link ImageAnalysis}. */
@Override
public void setTargetRotation(@NonNull Long identifier, @NonNull Long rotation) {
ImageAnalysis imageAnalysis = getImageAnalysisInstance(identifier);
imageAnalysis.setTargetRotation(rotation.intValue());
}
/**
* Retrieives the {@link ImageAnalysis} instance associated with the specified {@code identifier}.
*/
private ImageAnalysis getImageAnalysisInstance(@NonNull Long identifier) {
return Objects.requireNonNull(instanceManager.getInstance(identifier));
}
}
| packages/packages/camera/camera_android_camerax/android/src/main/java/io/flutter/plugins/camerax/ImageAnalysisHostApiImpl.java/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/android/src/main/java/io/flutter/plugins/camerax/ImageAnalysisHostApiImpl.java",
"repo_id": "packages",
"token_count": 1103
} | 938 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.camerax;
import static org.junit.Assert.assertEquals;
import static org.mockito.ArgumentMatchers.any;
import static org.mockito.ArgumentMatchers.eq;
import static org.mockito.Mockito.mock;
import static org.mockito.Mockito.verify;
import static org.mockito.Mockito.when;
import android.content.Context;
import androidx.camera.camera2.interop.Camera2CameraControl;
import androidx.camera.camera2.interop.CaptureRequestOptions;
import androidx.camera.core.CameraControl;
import com.google.common.util.concurrent.FutureCallback;
import com.google.common.util.concurrent.Futures;
import com.google.common.util.concurrent.ListenableFuture;
import org.junit.After;
import org.junit.Before;
import org.junit.Rule;
import org.junit.Test;
import org.mockito.ArgumentCaptor;
import org.mockito.Mock;
import org.mockito.MockedStatic;
import org.mockito.Mockito;
import org.mockito.junit.MockitoJUnit;
import org.mockito.junit.MockitoRule;
import org.mockito.stubbing.Answer;
public class Camera2CameraControlTest {
@Rule public MockitoRule mockitoRule = MockitoJUnit.rule();
@Mock public Camera2CameraControl mockCamera2CameraControl;
InstanceManager testInstanceManager;
@Before
public void setUp() {
testInstanceManager = InstanceManager.create(identifier -> {});
}
@After
public void tearDown() {
testInstanceManager.stopFinalizationListener();
}
@Test
public void create_createsInstanceFromCameraControlInstance() {
final Camera2CameraControlHostApiImpl hostApi =
new Camera2CameraControlHostApiImpl(testInstanceManager, mock(Context.class));
final long instanceIdentifier = 40;
final CameraControl mockCameraControl = mock(CameraControl.class);
final long cameraControlIdentifier = 29;
testInstanceManager.addDartCreatedInstance(mockCameraControl, cameraControlIdentifier);
try (MockedStatic<Camera2CameraControl> mockedCamera2CameraControl =
Mockito.mockStatic(Camera2CameraControl.class)) {
mockedCamera2CameraControl
.when(() -> Camera2CameraControl.from(mockCameraControl))
.thenAnswer((Answer<Camera2CameraControl>) invocation -> mockCamera2CameraControl);
hostApi.create(instanceIdentifier, cameraControlIdentifier);
assertEquals(testInstanceManager.getInstance(instanceIdentifier), mockCamera2CameraControl);
}
}
@Test
public void addCaptureRequestOptions_respondsAsExpectedToSuccessfulAndFailedAttempts() {
final Camera2CameraControlHostApiImpl hostApi =
new Camera2CameraControlHostApiImpl(testInstanceManager, mock(Context.class));
final long instanceIdentifier = 0;
final CaptureRequestOptions mockCaptureRequestOptions = mock(CaptureRequestOptions.class);
final long captureRequestOptionsIdentifier = 8;
testInstanceManager.addDartCreatedInstance(mockCamera2CameraControl, instanceIdentifier);
testInstanceManager.addDartCreatedInstance(
mockCaptureRequestOptions, captureRequestOptionsIdentifier);
try (MockedStatic<Futures> mockedFutures = Mockito.mockStatic(Futures.class)) {
@SuppressWarnings("unchecked")
final ListenableFuture<Void> addCaptureRequestOptionsFuture = mock(ListenableFuture.class);
when(mockCamera2CameraControl.addCaptureRequestOptions(mockCaptureRequestOptions))
.thenReturn(addCaptureRequestOptionsFuture);
@SuppressWarnings("unchecked")
final ArgumentCaptor<FutureCallback<Void>> futureCallbackCaptor =
ArgumentCaptor.forClass(FutureCallback.class);
// Test successfully adding capture request options.
@SuppressWarnings("unchecked")
final GeneratedCameraXLibrary.Result<Void> successfulMockResult =
mock(GeneratedCameraXLibrary.Result.class);
hostApi.addCaptureRequestOptions(
instanceIdentifier, captureRequestOptionsIdentifier, successfulMockResult);
mockedFutures.verify(
() ->
Futures.addCallback(
eq(addCaptureRequestOptionsFuture), futureCallbackCaptor.capture(), any()));
mockedFutures.clearInvocations();
FutureCallback<Void> successfulCallback = futureCallbackCaptor.getValue();
successfulCallback.onSuccess(mock(Void.class));
verify(successfulMockResult).success(null);
// Test failed attempt to add capture request options.
@SuppressWarnings("unchecked")
final GeneratedCameraXLibrary.Result<Void> failedMockResult =
mock(GeneratedCameraXLibrary.Result.class);
final Throwable testThrowable = new Throwable();
hostApi.addCaptureRequestOptions(
instanceIdentifier, captureRequestOptionsIdentifier, failedMockResult);
mockedFutures.verify(
() ->
Futures.addCallback(
eq(addCaptureRequestOptionsFuture), futureCallbackCaptor.capture(), any()));
FutureCallback<Void> failedCallback = futureCallbackCaptor.getValue();
failedCallback.onFailure(testThrowable);
verify(failedMockResult).error(testThrowable);
}
}
}
| packages/packages/camera/camera_android_camerax/android/src/test/java/io/flutter/plugins/camerax/Camera2CameraControlTest.java/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/android/src/test/java/io/flutter/plugins/camerax/Camera2CameraControlTest.java",
"repo_id": "packages",
"token_count": 1720
} | 939 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.camerax;
import static org.junit.Assert.assertEquals;
import static org.mockito.ArgumentMatchers.any;
import static org.mockito.ArgumentMatchers.eq;
import static org.mockito.Mockito.mock;
import static org.mockito.Mockito.verify;
import static org.mockito.Mockito.when;
import android.content.Context;
import android.view.Surface;
import androidx.camera.core.ImageAnalysis;
import androidx.camera.core.resolutionselector.ResolutionSelector;
import androidx.test.core.app.ApplicationProvider;
import io.flutter.plugin.common.BinaryMessenger;
import java.util.concurrent.Executor;
import org.junit.After;
import org.junit.Before;
import org.junit.Rule;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.mockito.Mock;
import org.mockito.junit.MockitoJUnit;
import org.mockito.junit.MockitoRule;
import org.robolectric.RobolectricTestRunner;
@RunWith(RobolectricTestRunner.class)
public class ImageAnalysisTest {
@Rule public MockitoRule mockitoRule = MockitoJUnit.rule();
@Mock public ImageAnalysis mockImageAnalysis;
@Mock public BinaryMessenger mockBinaryMessenger;
InstanceManager instanceManager;
private Context context;
@Before
public void setUp() {
instanceManager = InstanceManager.create(identifier -> {});
context = ApplicationProvider.getApplicationContext();
}
@After
public void tearDown() {
instanceManager.stopFinalizationListener();
}
@Test
public void hostApiCreate_createsExpectedImageAnalysisInstanceWithExpectedIdentifier() {
final ImageAnalysisHostApiImpl hostApi =
new ImageAnalysisHostApiImpl(mockBinaryMessenger, instanceManager, context);
final CameraXProxy mockCameraXProxy = mock(CameraXProxy.class);
final ImageAnalysis.Builder mockImageAnalysisBuilder = mock(ImageAnalysis.Builder.class);
final ResolutionSelector mockResolutionSelector = mock(ResolutionSelector.class);
final long instanceIdentifier = 0;
final long mockResolutionSelectorId = 25;
final int targetRotation = Surface.ROTATION_90;
hostApi.cameraXProxy = mockCameraXProxy;
instanceManager.addDartCreatedInstance(mockResolutionSelector, mockResolutionSelectorId);
when(mockCameraXProxy.createImageAnalysisBuilder()).thenReturn(mockImageAnalysisBuilder);
when(mockImageAnalysisBuilder.build()).thenReturn(mockImageAnalysis);
hostApi.create(instanceIdentifier, Long.valueOf(targetRotation), mockResolutionSelectorId);
verify(mockImageAnalysisBuilder).setTargetRotation(targetRotation);
verify(mockImageAnalysisBuilder).setResolutionSelector(mockResolutionSelector);
assertEquals(instanceManager.getInstance(instanceIdentifier), mockImageAnalysis);
}
@Test
public void setAnalyzer_makesCallToSetAnalyzerOnExpectedImageAnalysisInstance() {
final ImageAnalysisHostApiImpl hostApi =
new ImageAnalysisHostApiImpl(mockBinaryMessenger, instanceManager, context);
final ImageAnalysis.Analyzer mockAnalyzer = mock(ImageAnalysis.Analyzer.class);
final long analyzerIdentifier = 10;
final long instanceIdentifier = 94;
instanceManager.addDartCreatedInstance(mockAnalyzer, analyzerIdentifier);
instanceManager.addDartCreatedInstance(mockImageAnalysis, instanceIdentifier);
hostApi.setAnalyzer(instanceIdentifier, analyzerIdentifier);
verify(mockImageAnalysis).setAnalyzer(any(Executor.class), eq(mockAnalyzer));
}
@Test
public void clearAnalyzer_makesCallToClearAnalyzerOnExpectedImageAnalysisInstance() {
final ImageAnalysisHostApiImpl hostApi =
new ImageAnalysisHostApiImpl(mockBinaryMessenger, instanceManager, context);
final long instanceIdentifier = 22;
instanceManager.addDartCreatedInstance(mockImageAnalysis, instanceIdentifier);
hostApi.clearAnalyzer(instanceIdentifier);
verify(mockImageAnalysis).clearAnalyzer();
}
@Test
public void setTargetRotation_makesCallToSetTargetRotation() {
final ImageAnalysisHostApiImpl hostApi =
new ImageAnalysisHostApiImpl(mockBinaryMessenger, instanceManager, context);
final long instanceIdentifier = 32;
final int targetRotation = Surface.ROTATION_180;
instanceManager.addDartCreatedInstance(mockImageAnalysis, instanceIdentifier);
hostApi.setTargetRotation(instanceIdentifier, Long.valueOf(targetRotation));
verify(mockImageAnalysis).setTargetRotation(targetRotation);
}
}
| packages/packages/camera/camera_android_camerax/android/src/test/java/io/flutter/plugins/camerax/ImageAnalysisTest.java/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/android/src/test/java/io/flutter/plugins/camerax/ImageAnalysisTest.java",
"repo_id": "packages",
"token_count": 1401
} | 940 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.camerax;
import static org.junit.Assert.assertEquals;
import static org.mockito.Mockito.mock;
import static org.mockito.Mockito.when;
import androidx.camera.core.resolutionselector.AspectRatioStrategy;
import androidx.camera.core.resolutionselector.ResolutionSelector;
import androidx.camera.core.resolutionselector.ResolutionStrategy;
import org.junit.After;
import org.junit.Before;
import org.junit.Rule;
import org.junit.Test;
import org.mockito.Mock;
import org.mockito.junit.MockitoJUnit;
import org.mockito.junit.MockitoRule;
public class ResolutionSelectorTest {
@Rule public MockitoRule mockitoRule = MockitoJUnit.rule();
@Mock public ResolutionSelector mockResolutionSelector;
@Mock public ResolutionSelectorHostApiImpl.ResolutionSelectorProxy mockProxy;
InstanceManager instanceManager;
@Before
public void setUp() {
instanceManager = InstanceManager.create(identifier -> {});
}
@After
public void tearDown() {
instanceManager.stopFinalizationListener();
}
@Test
public void hostApiCreate_createsExpectedResolutionSelectorInstance() {
final ResolutionStrategy mockResolutionStrategy = mock(ResolutionStrategy.class);
final long resolutionStrategyIdentifier = 14;
instanceManager.addDartCreatedInstance(mockResolutionStrategy, resolutionStrategyIdentifier);
final AspectRatioStrategy mockAspectRatioStrategy = mock(AspectRatioStrategy.class);
final long aspectRatioStrategyIdentifier = 15;
instanceManager.addDartCreatedInstance(mockAspectRatioStrategy, aspectRatioStrategyIdentifier);
when(mockProxy.create(mockResolutionStrategy, mockAspectRatioStrategy))
.thenReturn(mockResolutionSelector);
final ResolutionSelectorHostApiImpl hostApi =
new ResolutionSelectorHostApiImpl(instanceManager, mockProxy);
final long instanceIdentifier = 0;
hostApi.create(instanceIdentifier, resolutionStrategyIdentifier, aspectRatioStrategyIdentifier);
assertEquals(instanceManager.getInstance(instanceIdentifier), mockResolutionSelector);
}
}
| packages/packages/camera/camera_android_camerax/android/src/test/java/io/flutter/plugins/camerax/ResolutionSelectorTest.java/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/android/src/test/java/io/flutter/plugins/camerax/ResolutionSelectorTest.java",
"repo_id": "packages",
"token_count": 689
} | 941 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// Autogenerated from Pigeon (v9.2.5), do not edit directly.
// See also: https://pub.dev/packages/pigeon
// ignore_for_file: public_member_api_docs, non_constant_identifier_names, avoid_as, unused_import, unnecessary_parenthesis, prefer_null_aware_operators, omit_local_variable_types, unused_shown_name, unnecessary_import
import 'dart:async';
import 'dart:typed_data' show Float64List, Int32List, Int64List, Uint8List;
import 'package:flutter/foundation.dart' show ReadBuffer, WriteBuffer;
import 'package:flutter/services.dart';
/// The states the camera can be in.
///
/// See https://developer.android.com/reference/androidx/camera/core/CameraState.Type.
enum CameraStateType {
closed,
closing,
open,
opening,
pendingOpen,
}
/// The types (T) properly wrapped to be used as a LiveData<T>.
///
/// If you need to add another type to support a type S to use a LiveData<S> in
/// this plugin, ensure the following is done on the Dart side:
///
/// * In `camera_android_camerax/lib/src/live_data.dart`, add new cases for S in
/// `_LiveDataHostApiImpl#getValueFromInstances` to get the current value of
/// type S from a LiveData<S> instance and in `LiveDataFlutterApiImpl#create`
/// to create the expected type of LiveData<S> when requested.
///
/// On the native side, ensure the following is done:
///
/// * Make sure `LiveDataHostApiImpl#getValue` is updated to properly return
/// identifiers for instances of type S.
/// * Update `ObserverFlutterApiWrapper#onChanged` to properly handle receiving
/// calls with instances of type S if a LiveData<S> instance is observed.
enum LiveDataSupportedType {
cameraState,
zoomState,
}
/// Video quality constraints that will be used by a QualitySelector to choose
/// an appropriate video resolution.
///
/// These are pre-defined quality constants that are universally used for video.
///
/// See https://developer.android.com/reference/androidx/camera/video/Quality.
enum VideoQuality {
SD,
HD,
FHD,
UHD,
lowest,
highest,
}
/// Fallback rules for selecting video resolution.
///
/// See https://developer.android.com/reference/androidx/camera/video/FallbackStrategy.
enum VideoResolutionFallbackRule {
higherQualityOrLowerThan,
higherQualityThan,
lowerQualityOrHigherThan,
lowerQualityThan,
}
/// The types of capture request options this plugin currently supports.
///
/// If you need to add another option to support, ensure the following is done
/// on the Dart side:
///
/// * In `camera_android_camerax/lib/src/capture_request_options.dart`, add new cases for this
/// option in `_CaptureRequestOptionsHostApiImpl#createFromInstances`
/// to create the expected Map entry of option key index and value to send to
/// the native side.
///
/// On the native side, ensure the following is done:
///
/// * Update `CaptureRequestOptionsHostApiImpl#create` to set the correct
/// `CaptureRequest` key with a valid value type for this option.
///
/// See https://developer.android.com/reference/android/hardware/camera2/CaptureRequest
/// for the sorts of capture request options that can be supported via CameraX's
/// interoperability with Camera2.
enum CaptureRequestKeySupportedType {
controlAeLock,
}
class ResolutionInfo {
ResolutionInfo({
required this.width,
required this.height,
});
int width;
int height;
Object encode() {
return <Object?>[
width,
height,
];
}
static ResolutionInfo decode(Object result) {
result as List<Object?>;
return ResolutionInfo(
width: result[0]! as int,
height: result[1]! as int,
);
}
}
class CameraPermissionsErrorData {
CameraPermissionsErrorData({
required this.errorCode,
required this.description,
});
String errorCode;
String description;
Object encode() {
return <Object?>[
errorCode,
description,
];
}
static CameraPermissionsErrorData decode(Object result) {
result as List<Object?>;
return CameraPermissionsErrorData(
errorCode: result[0]! as String,
description: result[1]! as String,
);
}
}
class CameraStateTypeData {
CameraStateTypeData({
required this.value,
});
CameraStateType value;
Object encode() {
return <Object?>[
value.index,
];
}
static CameraStateTypeData decode(Object result) {
result as List<Object?>;
return CameraStateTypeData(
value: CameraStateType.values[result[0]! as int],
);
}
}
class LiveDataSupportedTypeData {
LiveDataSupportedTypeData({
required this.value,
});
LiveDataSupportedType value;
Object encode() {
return <Object?>[
value.index,
];
}
static LiveDataSupportedTypeData decode(Object result) {
result as List<Object?>;
return LiveDataSupportedTypeData(
value: LiveDataSupportedType.values[result[0]! as int],
);
}
}
class ExposureCompensationRange {
ExposureCompensationRange({
required this.minCompensation,
required this.maxCompensation,
});
int minCompensation;
int maxCompensation;
Object encode() {
return <Object?>[
minCompensation,
maxCompensation,
];
}
static ExposureCompensationRange decode(Object result) {
result as List<Object?>;
return ExposureCompensationRange(
minCompensation: result[0]! as int,
maxCompensation: result[1]! as int,
);
}
}
/// Convenience class for sending lists of [Quality]s.
class VideoQualityData {
VideoQualityData({
required this.quality,
});
VideoQuality quality;
Object encode() {
return <Object?>[
quality.index,
];
}
static VideoQualityData decode(Object result) {
result as List<Object?>;
return VideoQualityData(
quality: VideoQuality.values[result[0]! as int],
);
}
}
/// Convenience class for building [FocusMeteringAction]s with multiple metering
/// points.
class MeteringPointInfo {
MeteringPointInfo({
required this.meteringPointId,
this.meteringMode,
});
/// InstanceManager ID for a [MeteringPoint].
int meteringPointId;
/// The metering mode of the [MeteringPoint] whose ID is [meteringPointId].
///
/// Metering mode should be one of the [FocusMeteringAction] constants.
int? meteringMode;
Object encode() {
return <Object?>[
meteringPointId,
meteringMode,
];
}
static MeteringPointInfo decode(Object result) {
result as List<Object?>;
return MeteringPointInfo(
meteringPointId: result[0]! as int,
meteringMode: result[1] as int?,
);
}
}
class InstanceManagerHostApi {
/// Constructor for [InstanceManagerHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
InstanceManagerHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
/// Clear the native `InstanceManager`.
///
/// This is typically only used after a hot restart.
Future<void> clear() async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.InstanceManagerHostApi.clear', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(null) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
class JavaObjectHostApi {
/// Constructor for [JavaObjectHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
JavaObjectHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> dispose(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.JavaObjectHostApi.dispose', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
abstract class JavaObjectFlutterApi {
static const MessageCodec<Object?> codec = StandardMessageCodec();
void dispose(int identifier);
static void setup(JavaObjectFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.JavaObjectFlutterApi.dispose', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.JavaObjectFlutterApi.dispose was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.JavaObjectFlutterApi.dispose was null, expected non-null int.');
api.dispose(arg_identifier!);
return;
});
}
}
}
}
class CameraInfoHostApi {
/// Constructor for [CameraInfoHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
CameraInfoHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<int> getSensorRotationDegrees(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.CameraInfoHostApi.getSensorRotationDegrees', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as int?)!;
}
}
Future<int> getCameraState(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.CameraInfoHostApi.getCameraState', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as int?)!;
}
}
Future<int> getExposureState(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.CameraInfoHostApi.getExposureState', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as int?)!;
}
}
Future<int> getZoomState(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.CameraInfoHostApi.getZoomState', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as int?)!;
}
}
}
abstract class CameraInfoFlutterApi {
static const MessageCodec<Object?> codec = StandardMessageCodec();
void create(int identifier);
static void setup(CameraInfoFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.CameraInfoFlutterApi.create', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.CameraInfoFlutterApi.create was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.CameraInfoFlutterApi.create was null, expected non-null int.');
api.create(arg_identifier!);
return;
});
}
}
}
}
class CameraSelectorHostApi {
/// Constructor for [CameraSelectorHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
CameraSelectorHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> create(int arg_identifier, int? arg_lensFacing) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.CameraSelectorHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_lensFacing]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<List<int?>> filter(
int arg_identifier, List<int?> arg_cameraInfoIds) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.CameraSelectorHostApi.filter', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_cameraInfoIds]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as List<Object?>?)!.cast<int?>();
}
}
}
abstract class CameraSelectorFlutterApi {
static const MessageCodec<Object?> codec = StandardMessageCodec();
void create(int identifier, int? lensFacing);
static void setup(CameraSelectorFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.CameraSelectorFlutterApi.create', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.CameraSelectorFlutterApi.create was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.CameraSelectorFlutterApi.create was null, expected non-null int.');
final int? arg_lensFacing = (args[1] as int?);
api.create(arg_identifier!, arg_lensFacing);
return;
});
}
}
}
}
class ProcessCameraProviderHostApi {
/// Constructor for [ProcessCameraProviderHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
ProcessCameraProviderHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<int> getInstance() async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ProcessCameraProviderHostApi.getInstance', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(null) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as int?)!;
}
}
Future<List<int?>> getAvailableCameraInfos(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ProcessCameraProviderHostApi.getAvailableCameraInfos',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as List<Object?>?)!.cast<int?>();
}
}
Future<int> bindToLifecycle(int arg_identifier,
int arg_cameraSelectorIdentifier, List<int?> arg_useCaseIds) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ProcessCameraProviderHostApi.bindToLifecycle',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(<Object?>[
arg_identifier,
arg_cameraSelectorIdentifier,
arg_useCaseIds
]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as int?)!;
}
}
Future<bool> isBound(int arg_identifier, int arg_useCaseIdentifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ProcessCameraProviderHostApi.isBound', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier, arg_useCaseIdentifier])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as bool?)!;
}
}
Future<void> unbind(int arg_identifier, List<int?> arg_useCaseIds) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ProcessCameraProviderHostApi.unbind', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_useCaseIds]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> unbindAll(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ProcessCameraProviderHostApi.unbindAll', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
abstract class ProcessCameraProviderFlutterApi {
static const MessageCodec<Object?> codec = StandardMessageCodec();
void create(int identifier);
static void setup(ProcessCameraProviderFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ProcessCameraProviderFlutterApi.create', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.ProcessCameraProviderFlutterApi.create was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.ProcessCameraProviderFlutterApi.create was null, expected non-null int.');
api.create(arg_identifier!);
return;
});
}
}
}
}
class CameraHostApi {
/// Constructor for [CameraHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
CameraHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<int> getCameraInfo(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.CameraHostApi.getCameraInfo', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as int?)!;
}
}
Future<int> getCameraControl(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.CameraHostApi.getCameraControl', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as int?)!;
}
}
}
abstract class CameraFlutterApi {
static const MessageCodec<Object?> codec = StandardMessageCodec();
void create(int identifier);
static void setup(CameraFlutterApi? api, {BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.CameraFlutterApi.create', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.CameraFlutterApi.create was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.CameraFlutterApi.create was null, expected non-null int.');
api.create(arg_identifier!);
return;
});
}
}
}
}
class _SystemServicesHostApiCodec extends StandardMessageCodec {
const _SystemServicesHostApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is CameraPermissionsErrorData) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return CameraPermissionsErrorData.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
class SystemServicesHostApi {
/// Constructor for [SystemServicesHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
SystemServicesHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = _SystemServicesHostApiCodec();
Future<CameraPermissionsErrorData?> requestCameraPermissions(
bool arg_enableAudio) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.SystemServicesHostApi.requestCameraPermissions',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_enableAudio]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return (replyList[0] as CameraPermissionsErrorData?);
}
}
Future<String> getTempFilePath(String arg_prefix, String arg_suffix) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.SystemServicesHostApi.getTempFilePath', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_prefix, arg_suffix]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as String?)!;
}
}
}
abstract class SystemServicesFlutterApi {
static const MessageCodec<Object?> codec = StandardMessageCodec();
void onCameraError(String errorDescription);
static void setup(SystemServicesFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.SystemServicesFlutterApi.onCameraError', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.SystemServicesFlutterApi.onCameraError was null.');
final List<Object?> args = (message as List<Object?>?)!;
final String? arg_errorDescription = (args[0] as String?);
assert(arg_errorDescription != null,
'Argument for dev.flutter.pigeon.SystemServicesFlutterApi.onCameraError was null, expected non-null String.');
api.onCameraError(arg_errorDescription!);
return;
});
}
}
}
}
class DeviceOrientationManagerHostApi {
/// Constructor for [DeviceOrientationManagerHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
DeviceOrientationManagerHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> startListeningForDeviceOrientationChange(
bool arg_isFrontFacing, int arg_sensorOrientation) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.DeviceOrientationManagerHostApi.startListeningForDeviceOrientationChange',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_isFrontFacing, arg_sensorOrientation])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> stopListeningForDeviceOrientationChange() async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.DeviceOrientationManagerHostApi.stopListeningForDeviceOrientationChange',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(null) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<int> getDefaultDisplayRotation() async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.DeviceOrientationManagerHostApi.getDefaultDisplayRotation',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(null) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as int?)!;
}
}
}
abstract class DeviceOrientationManagerFlutterApi {
static const MessageCodec<Object?> codec = StandardMessageCodec();
void onDeviceOrientationChanged(String orientation);
static void setup(DeviceOrientationManagerFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.DeviceOrientationManagerFlutterApi.onDeviceOrientationChanged',
codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.DeviceOrientationManagerFlutterApi.onDeviceOrientationChanged was null.');
final List<Object?> args = (message as List<Object?>?)!;
final String? arg_orientation = (args[0] as String?);
assert(arg_orientation != null,
'Argument for dev.flutter.pigeon.DeviceOrientationManagerFlutterApi.onDeviceOrientationChanged was null, expected non-null String.');
api.onDeviceOrientationChanged(arg_orientation!);
return;
});
}
}
}
}
class _PreviewHostApiCodec extends StandardMessageCodec {
const _PreviewHostApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is ResolutionInfo) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return ResolutionInfo.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
class PreviewHostApi {
/// Constructor for [PreviewHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
PreviewHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = _PreviewHostApiCodec();
Future<void> create(int arg_identifier, int? arg_rotation,
int? arg_resolutionSelectorId) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.PreviewHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(
<Object?>[arg_identifier, arg_rotation, arg_resolutionSelectorId])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<int> setSurfaceProvider(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.PreviewHostApi.setSurfaceProvider', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as int?)!;
}
}
Future<void> releaseFlutterSurfaceTexture() async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.PreviewHostApi.releaseFlutterSurfaceTexture', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(null) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<ResolutionInfo> getResolutionInfo(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.PreviewHostApi.getResolutionInfo', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as ResolutionInfo?)!;
}
}
Future<void> setTargetRotation(int arg_identifier, int arg_rotation) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.PreviewHostApi.setTargetRotation', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_rotation]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
class VideoCaptureHostApi {
/// Constructor for [VideoCaptureHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
VideoCaptureHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<int> withOutput(int arg_videoOutputId) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.VideoCaptureHostApi.withOutput', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_videoOutputId]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as int?)!;
}
}
Future<int> getOutput(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.VideoCaptureHostApi.getOutput', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as int?)!;
}
}
Future<void> setTargetRotation(int arg_identifier, int arg_rotation) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.VideoCaptureHostApi.setTargetRotation', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_rotation]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
abstract class VideoCaptureFlutterApi {
static const MessageCodec<Object?> codec = StandardMessageCodec();
void create(int identifier);
static void setup(VideoCaptureFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.VideoCaptureFlutterApi.create', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.VideoCaptureFlutterApi.create was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.VideoCaptureFlutterApi.create was null, expected non-null int.');
api.create(arg_identifier!);
return;
});
}
}
}
}
class RecorderHostApi {
/// Constructor for [RecorderHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
RecorderHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> create(int arg_identifier, int? arg_aspectRatio,
int? arg_bitRate, int? arg_qualitySelectorId) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.RecorderHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(<Object?>[
arg_identifier,
arg_aspectRatio,
arg_bitRate,
arg_qualitySelectorId
]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<int> getAspectRatio(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.RecorderHostApi.getAspectRatio', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as int?)!;
}
}
Future<int> getTargetVideoEncodingBitRate(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.RecorderHostApi.getTargetVideoEncodingBitRate',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as int?)!;
}
}
Future<int> prepareRecording(int arg_identifier, String arg_path) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.RecorderHostApi.prepareRecording', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_path]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as int?)!;
}
}
}
abstract class RecorderFlutterApi {
static const MessageCodec<Object?> codec = StandardMessageCodec();
void create(int identifier, int? aspectRatio, int? bitRate);
static void setup(RecorderFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.RecorderFlutterApi.create', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.RecorderFlutterApi.create was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.RecorderFlutterApi.create was null, expected non-null int.');
final int? arg_aspectRatio = (args[1] as int?);
final int? arg_bitRate = (args[2] as int?);
api.create(arg_identifier!, arg_aspectRatio, arg_bitRate);
return;
});
}
}
}
}
class PendingRecordingHostApi {
/// Constructor for [PendingRecordingHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
PendingRecordingHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<int> start(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.PendingRecordingHostApi.start', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as int?)!;
}
}
}
abstract class PendingRecordingFlutterApi {
static const MessageCodec<Object?> codec = StandardMessageCodec();
void create(int identifier);
static void setup(PendingRecordingFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.PendingRecordingFlutterApi.create', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.PendingRecordingFlutterApi.create was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.PendingRecordingFlutterApi.create was null, expected non-null int.');
api.create(arg_identifier!);
return;
});
}
}
}
}
class RecordingHostApi {
/// Constructor for [RecordingHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
RecordingHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> close(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.RecordingHostApi.close', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> pause(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.RecordingHostApi.pause', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> resume(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.RecordingHostApi.resume', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> stop(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.RecordingHostApi.stop', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
abstract class RecordingFlutterApi {
static const MessageCodec<Object?> codec = StandardMessageCodec();
void create(int identifier);
static void setup(RecordingFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.RecordingFlutterApi.create', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.RecordingFlutterApi.create was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.RecordingFlutterApi.create was null, expected non-null int.');
api.create(arg_identifier!);
return;
});
}
}
}
}
class ImageCaptureHostApi {
/// Constructor for [ImageCaptureHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
ImageCaptureHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> create(int arg_identifier, int? arg_targetRotation,
int? arg_flashMode, int? arg_resolutionSelectorId) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ImageCaptureHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(<Object?>[
arg_identifier,
arg_targetRotation,
arg_flashMode,
arg_resolutionSelectorId
]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> setFlashMode(int arg_identifier, int arg_flashMode) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ImageCaptureHostApi.setFlashMode', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_flashMode]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<String> takePicture(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ImageCaptureHostApi.takePicture', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as String?)!;
}
}
Future<void> setTargetRotation(int arg_identifier, int arg_rotation) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ImageCaptureHostApi.setTargetRotation', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_rotation]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
class _ResolutionStrategyHostApiCodec extends StandardMessageCodec {
const _ResolutionStrategyHostApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is ResolutionInfo) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return ResolutionInfo.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
class ResolutionStrategyHostApi {
/// Constructor for [ResolutionStrategyHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
ResolutionStrategyHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = _ResolutionStrategyHostApiCodec();
Future<void> create(int arg_identifier, ResolutionInfo? arg_boundSize,
int? arg_fallbackRule) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ResolutionStrategyHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_boundSize, arg_fallbackRule])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
class ResolutionSelectorHostApi {
/// Constructor for [ResolutionSelectorHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
ResolutionSelectorHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> create(int arg_identifier, int? arg_resolutionStrategyIdentifier,
int? arg_aspectRatioStrategyIdentifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ResolutionSelectorHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(<Object?>[
arg_identifier,
arg_resolutionStrategyIdentifier,
arg_aspectRatioStrategyIdentifier
]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
class AspectRatioStrategyHostApi {
/// Constructor for [AspectRatioStrategyHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
AspectRatioStrategyHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> create(int arg_identifier, int arg_preferredAspectRatio,
int arg_fallbackRule) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.AspectRatioStrategyHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(<Object?>[
arg_identifier,
arg_preferredAspectRatio,
arg_fallbackRule
]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
class _CameraStateFlutterApiCodec extends StandardMessageCodec {
const _CameraStateFlutterApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is CameraStateTypeData) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return CameraStateTypeData.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
abstract class CameraStateFlutterApi {
static const MessageCodec<Object?> codec = _CameraStateFlutterApiCodec();
void create(int identifier, CameraStateTypeData type, int? errorIdentifier);
static void setup(CameraStateFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.CameraStateFlutterApi.create', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.CameraStateFlutterApi.create was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.CameraStateFlutterApi.create was null, expected non-null int.');
final CameraStateTypeData? arg_type =
(args[1] as CameraStateTypeData?);
assert(arg_type != null,
'Argument for dev.flutter.pigeon.CameraStateFlutterApi.create was null, expected non-null CameraStateTypeData.');
final int? arg_errorIdentifier = (args[2] as int?);
api.create(arg_identifier!, arg_type!, arg_errorIdentifier);
return;
});
}
}
}
}
class _ExposureStateFlutterApiCodec extends StandardMessageCodec {
const _ExposureStateFlutterApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is ExposureCompensationRange) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return ExposureCompensationRange.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
abstract class ExposureStateFlutterApi {
static const MessageCodec<Object?> codec = _ExposureStateFlutterApiCodec();
void create(
int identifier,
ExposureCompensationRange exposureCompensationRange,
double exposureCompensationStep);
static void setup(ExposureStateFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ExposureStateFlutterApi.create', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.ExposureStateFlutterApi.create was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.ExposureStateFlutterApi.create was null, expected non-null int.');
final ExposureCompensationRange? arg_exposureCompensationRange =
(args[1] as ExposureCompensationRange?);
assert(arg_exposureCompensationRange != null,
'Argument for dev.flutter.pigeon.ExposureStateFlutterApi.create was null, expected non-null ExposureCompensationRange.');
final double? arg_exposureCompensationStep = (args[2] as double?);
assert(arg_exposureCompensationStep != null,
'Argument for dev.flutter.pigeon.ExposureStateFlutterApi.create was null, expected non-null double.');
api.create(arg_identifier!, arg_exposureCompensationRange!,
arg_exposureCompensationStep!);
return;
});
}
}
}
}
abstract class ZoomStateFlutterApi {
static const MessageCodec<Object?> codec = StandardMessageCodec();
void create(int identifier, double minZoomRatio, double maxZoomRatio);
static void setup(ZoomStateFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ZoomStateFlutterApi.create', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.ZoomStateFlutterApi.create was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.ZoomStateFlutterApi.create was null, expected non-null int.');
final double? arg_minZoomRatio = (args[1] as double?);
assert(arg_minZoomRatio != null,
'Argument for dev.flutter.pigeon.ZoomStateFlutterApi.create was null, expected non-null double.');
final double? arg_maxZoomRatio = (args[2] as double?);
assert(arg_maxZoomRatio != null,
'Argument for dev.flutter.pigeon.ZoomStateFlutterApi.create was null, expected non-null double.');
api.create(arg_identifier!, arg_minZoomRatio!, arg_maxZoomRatio!);
return;
});
}
}
}
}
class ImageAnalysisHostApi {
/// Constructor for [ImageAnalysisHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
ImageAnalysisHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> create(int arg_identifier, int? arg_targetRotation,
int? arg_resolutionSelectorId) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ImageAnalysisHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(<Object?>[
arg_identifier,
arg_targetRotation,
arg_resolutionSelectorId
]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> setAnalyzer(
int arg_identifier, int arg_analyzerIdentifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ImageAnalysisHostApi.setAnalyzer', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier, arg_analyzerIdentifier])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> clearAnalyzer(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ImageAnalysisHostApi.clearAnalyzer', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> setTargetRotation(int arg_identifier, int arg_rotation) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ImageAnalysisHostApi.setTargetRotation', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_rotation]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
class AnalyzerHostApi {
/// Constructor for [AnalyzerHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
AnalyzerHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> create(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.AnalyzerHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
class ObserverHostApi {
/// Constructor for [ObserverHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
ObserverHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> create(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ObserverHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
abstract class ObserverFlutterApi {
static const MessageCodec<Object?> codec = StandardMessageCodec();
void onChanged(int identifier, int valueIdentifier);
static void setup(ObserverFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ObserverFlutterApi.onChanged', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.ObserverFlutterApi.onChanged was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.ObserverFlutterApi.onChanged was null, expected non-null int.');
final int? arg_valueIdentifier = (args[1] as int?);
assert(arg_valueIdentifier != null,
'Argument for dev.flutter.pigeon.ObserverFlutterApi.onChanged was null, expected non-null int.');
api.onChanged(arg_identifier!, arg_valueIdentifier!);
return;
});
}
}
}
}
abstract class CameraStateErrorFlutterApi {
static const MessageCodec<Object?> codec = StandardMessageCodec();
void create(int identifier, int code);
static void setup(CameraStateErrorFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.CameraStateErrorFlutterApi.create', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.CameraStateErrorFlutterApi.create was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.CameraStateErrorFlutterApi.create was null, expected non-null int.');
final int? arg_code = (args[1] as int?);
assert(arg_code != null,
'Argument for dev.flutter.pigeon.CameraStateErrorFlutterApi.create was null, expected non-null int.');
api.create(arg_identifier!, arg_code!);
return;
});
}
}
}
}
class _LiveDataHostApiCodec extends StandardMessageCodec {
const _LiveDataHostApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is LiveDataSupportedTypeData) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return LiveDataSupportedTypeData.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
class LiveDataHostApi {
/// Constructor for [LiveDataHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
LiveDataHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = _LiveDataHostApiCodec();
Future<void> observe(int arg_identifier, int arg_observerIdentifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.LiveDataHostApi.observe', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier, arg_observerIdentifier])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> removeObservers(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.LiveDataHostApi.removeObservers', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<int?> getValue(
int arg_identifier, LiveDataSupportedTypeData arg_type) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.LiveDataHostApi.getValue', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_type]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return (replyList[0] as int?);
}
}
}
class _LiveDataFlutterApiCodec extends StandardMessageCodec {
const _LiveDataFlutterApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is LiveDataSupportedTypeData) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return LiveDataSupportedTypeData.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
abstract class LiveDataFlutterApi {
static const MessageCodec<Object?> codec = _LiveDataFlutterApiCodec();
void create(int identifier, LiveDataSupportedTypeData type);
static void setup(LiveDataFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.LiveDataFlutterApi.create', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.LiveDataFlutterApi.create was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.LiveDataFlutterApi.create was null, expected non-null int.');
final LiveDataSupportedTypeData? arg_type =
(args[1] as LiveDataSupportedTypeData?);
assert(arg_type != null,
'Argument for dev.flutter.pigeon.LiveDataFlutterApi.create was null, expected non-null LiveDataSupportedTypeData.');
api.create(arg_identifier!, arg_type!);
return;
});
}
}
}
}
abstract class AnalyzerFlutterApi {
static const MessageCodec<Object?> codec = StandardMessageCodec();
void create(int identifier);
void analyze(int identifier, int imageProxyIdentifier);
static void setup(AnalyzerFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.AnalyzerFlutterApi.create', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.AnalyzerFlutterApi.create was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.AnalyzerFlutterApi.create was null, expected non-null int.');
api.create(arg_identifier!);
return;
});
}
}
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.AnalyzerFlutterApi.analyze', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.AnalyzerFlutterApi.analyze was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.AnalyzerFlutterApi.analyze was null, expected non-null int.');
final int? arg_imageProxyIdentifier = (args[1] as int?);
assert(arg_imageProxyIdentifier != null,
'Argument for dev.flutter.pigeon.AnalyzerFlutterApi.analyze was null, expected non-null int.');
api.analyze(arg_identifier!, arg_imageProxyIdentifier!);
return;
});
}
}
}
}
class ImageProxyHostApi {
/// Constructor for [ImageProxyHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
ImageProxyHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<List<int?>> getPlanes(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ImageProxyHostApi.getPlanes', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as List<Object?>?)!.cast<int?>();
}
}
Future<void> close(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ImageProxyHostApi.close', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
abstract class ImageProxyFlutterApi {
static const MessageCodec<Object?> codec = StandardMessageCodec();
void create(int identifier, int format, int height, int width);
static void setup(ImageProxyFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ImageProxyFlutterApi.create', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.ImageProxyFlutterApi.create was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.ImageProxyFlutterApi.create was null, expected non-null int.');
final int? arg_format = (args[1] as int?);
assert(arg_format != null,
'Argument for dev.flutter.pigeon.ImageProxyFlutterApi.create was null, expected non-null int.');
final int? arg_height = (args[2] as int?);
assert(arg_height != null,
'Argument for dev.flutter.pigeon.ImageProxyFlutterApi.create was null, expected non-null int.');
final int? arg_width = (args[3] as int?);
assert(arg_width != null,
'Argument for dev.flutter.pigeon.ImageProxyFlutterApi.create was null, expected non-null int.');
api.create(arg_identifier!, arg_format!, arg_height!, arg_width!);
return;
});
}
}
}
}
abstract class PlaneProxyFlutterApi {
static const MessageCodec<Object?> codec = StandardMessageCodec();
void create(int identifier, Uint8List buffer, int pixelStride, int rowStride);
static void setup(PlaneProxyFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.PlaneProxyFlutterApi.create', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.PlaneProxyFlutterApi.create was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.PlaneProxyFlutterApi.create was null, expected non-null int.');
final Uint8List? arg_buffer = (args[1] as Uint8List?);
assert(arg_buffer != null,
'Argument for dev.flutter.pigeon.PlaneProxyFlutterApi.create was null, expected non-null Uint8List.');
final int? arg_pixelStride = (args[2] as int?);
assert(arg_pixelStride != null,
'Argument for dev.flutter.pigeon.PlaneProxyFlutterApi.create was null, expected non-null int.');
final int? arg_rowStride = (args[3] as int?);
assert(arg_rowStride != null,
'Argument for dev.flutter.pigeon.PlaneProxyFlutterApi.create was null, expected non-null int.');
api.create(
arg_identifier!, arg_buffer!, arg_pixelStride!, arg_rowStride!);
return;
});
}
}
}
}
class _QualitySelectorHostApiCodec extends StandardMessageCodec {
const _QualitySelectorHostApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is ResolutionInfo) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else if (value is VideoQualityData) {
buffer.putUint8(129);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return ResolutionInfo.decode(readValue(buffer)!);
case 129:
return VideoQualityData.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
class QualitySelectorHostApi {
/// Constructor for [QualitySelectorHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
QualitySelectorHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = _QualitySelectorHostApiCodec();
Future<void> create(
int arg_identifier,
List<VideoQualityData?> arg_videoQualityDataList,
int? arg_fallbackStrategyId) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.QualitySelectorHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(<Object?>[
arg_identifier,
arg_videoQualityDataList,
arg_fallbackStrategyId
]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<ResolutionInfo> getResolution(
int arg_cameraInfoId, VideoQuality arg_quality) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.QualitySelectorHostApi.getResolution', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_cameraInfoId, arg_quality.index]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as ResolutionInfo?)!;
}
}
}
class FallbackStrategyHostApi {
/// Constructor for [FallbackStrategyHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
FallbackStrategyHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> create(int arg_identifier, VideoQuality arg_quality,
VideoResolutionFallbackRule arg_fallbackRule) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.FallbackStrategyHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(<Object?>[
arg_identifier,
arg_quality.index,
arg_fallbackRule.index
]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
class CameraControlHostApi {
/// Constructor for [CameraControlHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
CameraControlHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> enableTorch(int arg_identifier, bool arg_torch) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.CameraControlHostApi.enableTorch', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_torch]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> setZoomRatio(int arg_identifier, double arg_ratio) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.CameraControlHostApi.setZoomRatio', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_ratio]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<int?> startFocusAndMetering(
int arg_identifier, int arg_focusMeteringActionId) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.CameraControlHostApi.startFocusAndMetering', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier, arg_focusMeteringActionId])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return (replyList[0] as int?);
}
}
Future<void> cancelFocusAndMetering(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.CameraControlHostApi.cancelFocusAndMetering', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<int?> setExposureCompensationIndex(
int arg_identifier, int arg_index) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.CameraControlHostApi.setExposureCompensationIndex',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_index]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return (replyList[0] as int?);
}
}
}
abstract class CameraControlFlutterApi {
static const MessageCodec<Object?> codec = StandardMessageCodec();
void create(int identifier);
static void setup(CameraControlFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.CameraControlFlutterApi.create', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.CameraControlFlutterApi.create was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.CameraControlFlutterApi.create was null, expected non-null int.');
api.create(arg_identifier!);
return;
});
}
}
}
}
class _FocusMeteringActionHostApiCodec extends StandardMessageCodec {
const _FocusMeteringActionHostApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is MeteringPointInfo) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return MeteringPointInfo.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
class FocusMeteringActionHostApi {
/// Constructor for [FocusMeteringActionHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
FocusMeteringActionHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = _FocusMeteringActionHostApiCodec();
Future<void> create(
int arg_identifier,
List<MeteringPointInfo?> arg_meteringPointInfos,
bool? arg_disableAutoCancel) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.FocusMeteringActionHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(<Object?>[
arg_identifier,
arg_meteringPointInfos,
arg_disableAutoCancel
]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
class FocusMeteringResultHostApi {
/// Constructor for [FocusMeteringResultHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
FocusMeteringResultHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<bool> isFocusSuccessful(int arg_identifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.FocusMeteringResultHostApi.isFocusSuccessful',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList =
await channel.send(<Object?>[arg_identifier]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as bool?)!;
}
}
}
abstract class FocusMeteringResultFlutterApi {
static const MessageCodec<Object?> codec = StandardMessageCodec();
void create(int identifier);
static void setup(FocusMeteringResultFlutterApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.FocusMeteringResultFlutterApi.create', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
channel.setMessageHandler(null);
} else {
channel.setMessageHandler((Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.FocusMeteringResultFlutterApi.create was null.');
final List<Object?> args = (message as List<Object?>?)!;
final int? arg_identifier = (args[0] as int?);
assert(arg_identifier != null,
'Argument for dev.flutter.pigeon.FocusMeteringResultFlutterApi.create was null, expected non-null int.');
api.create(arg_identifier!);
return;
});
}
}
}
}
class MeteringPointHostApi {
/// Constructor for [MeteringPointHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
MeteringPointHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> create(int arg_identifier, double arg_x, double arg_y,
double? arg_size, int arg_cameraInfoId) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.MeteringPointHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(
<Object?>[arg_identifier, arg_x, arg_y, arg_size, arg_cameraInfoId])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<double> getDefaultPointSize() async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.MeteringPointHostApi.getDefaultPointSize', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(null) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else if (replyList[0] == null) {
throw PlatformException(
code: 'null-error',
message: 'Host platform returned null value for non-null return value.',
);
} else {
return (replyList[0] as double?)!;
}
}
}
class _CaptureRequestOptionsHostApiCodec extends StandardMessageCodec {
const _CaptureRequestOptionsHostApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is CameraPermissionsErrorData) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else if (value is CameraStateTypeData) {
buffer.putUint8(129);
writeValue(buffer, value.encode());
} else if (value is ExposureCompensationRange) {
buffer.putUint8(130);
writeValue(buffer, value.encode());
} else if (value is LiveDataSupportedTypeData) {
buffer.putUint8(131);
writeValue(buffer, value.encode());
} else if (value is MeteringPointInfo) {
buffer.putUint8(132);
writeValue(buffer, value.encode());
} else if (value is ResolutionInfo) {
buffer.putUint8(133);
writeValue(buffer, value.encode());
} else if (value is VideoQualityData) {
buffer.putUint8(134);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return CameraPermissionsErrorData.decode(readValue(buffer)!);
case 129:
return CameraStateTypeData.decode(readValue(buffer)!);
case 130:
return ExposureCompensationRange.decode(readValue(buffer)!);
case 131:
return LiveDataSupportedTypeData.decode(readValue(buffer)!);
case 132:
return MeteringPointInfo.decode(readValue(buffer)!);
case 133:
return ResolutionInfo.decode(readValue(buffer)!);
case 134:
return VideoQualityData.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
class CaptureRequestOptionsHostApi {
/// Constructor for [CaptureRequestOptionsHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
CaptureRequestOptionsHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec =
_CaptureRequestOptionsHostApiCodec();
Future<void> create(
int arg_identifier, Map<int?, Object?> arg_options) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.CaptureRequestOptionsHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_options]) as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
class Camera2CameraControlHostApi {
/// Constructor for [Camera2CameraControlHostApi]. The [binaryMessenger] named argument is
/// available for dependency injection. If it is left null, the default
/// BinaryMessenger will be used which routes to the host platform.
Camera2CameraControlHostApi({BinaryMessenger? binaryMessenger})
: _binaryMessenger = binaryMessenger;
final BinaryMessenger? _binaryMessenger;
static const MessageCodec<Object?> codec = StandardMessageCodec();
Future<void> create(
int arg_identifier, int arg_cameraControlIdentifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.Camera2CameraControlHostApi.create', codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel
.send(<Object?>[arg_identifier, arg_cameraControlIdentifier])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
Future<void> addCaptureRequestOptions(
int arg_identifier, int arg_captureRequestOptionsIdentifier) async {
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.Camera2CameraControlHostApi.addCaptureRequestOptions',
codec,
binaryMessenger: _binaryMessenger);
final List<Object?>? replyList = await channel.send(
<Object?>[arg_identifier, arg_captureRequestOptionsIdentifier])
as List<Object?>?;
if (replyList == null) {
throw PlatformException(
code: 'channel-error',
message: 'Unable to establish connection on channel.',
);
} else if (replyList.length > 1) {
throw PlatformException(
code: replyList[0]! as String,
message: replyList[1] as String?,
details: replyList[2],
);
} else {
return;
}
}
}
| packages/packages/camera/camera_android_camerax/lib/src/camerax_library.g.dart/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/lib/src/camerax_library.g.dart",
"repo_id": "packages",
"token_count": 45845
} | 942 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart' show BinaryMessenger;
import 'package:meta/meta.dart' show immutable;
import 'android_camera_camerax_flutter_api_impls.dart';
import 'camerax_library.g.dart';
import 'instance_manager.dart';
import 'java_object.dart';
import 'recording.dart';
/// Dart wrapping of PendingRecording CameraX class.
///
/// See https://developer.android.com/reference/androidx/camera/video/PendingRecording
@immutable
class PendingRecording extends JavaObject {
/// Creates a [PendingRecording] that is not automatically attached to
/// a native object.
PendingRecording.detached(
{BinaryMessenger? binaryMessenger, InstanceManager? instanceManager})
: super.detached(
binaryMessenger: binaryMessenger,
instanceManager: instanceManager) {
_api = PendingRecordingHostApiImpl(
binaryMessenger: binaryMessenger, instanceManager: instanceManager);
AndroidCameraXCameraFlutterApis.instance.ensureSetUp();
}
late final PendingRecordingHostApiImpl _api;
/// Starts the recording, making it an active recording.
Future<Recording> start() {
return _api.startFromInstance(this);
}
}
/// Host API implementation of [PendingRecording].
class PendingRecordingHostApiImpl extends PendingRecordingHostApi {
/// Constructs a PendingRecordingHostApiImpl.
PendingRecordingHostApiImpl(
{this.binaryMessenger, InstanceManager? instanceManager})
: super(binaryMessenger: binaryMessenger) {
this.instanceManager = instanceManager ?? JavaObject.globalInstanceManager;
}
/// Receives binary data across the Flutter platform barrier.
///
/// If it is null, the default BinaryMessenger will be used which routes to
/// the host platform.
final BinaryMessenger? binaryMessenger;
/// Maintains instances stored to communicate with native language objects.
late final InstanceManager instanceManager;
/// Starts the recording, making it an active recording.
Future<Recording> startFromInstance(PendingRecording pendingRecording) async {
int? instanceId = instanceManager.getIdentifier(pendingRecording);
instanceId ??= instanceManager.addDartCreatedInstance(pendingRecording,
onCopy: (PendingRecording original) {
return PendingRecording.detached(
binaryMessenger: binaryMessenger,
instanceManager: instanceManager,
);
});
return instanceManager
.getInstanceWithWeakReference(await start(instanceId))! as Recording;
}
}
/// Flutter API implementation of [PendingRecording].
class PendingRecordingFlutterApiImpl extends PendingRecordingFlutterApi {
/// Constructs a [PendingRecordingFlutterApiImpl].
PendingRecordingFlutterApiImpl({
this.binaryMessenger,
InstanceManager? instanceManager,
}) : instanceManager = instanceManager ?? JavaObject.globalInstanceManager;
/// Receives binary data across the Flutter platform barrier.
///
/// If it is null, the default BinaryMessenger will be used which routes to
/// the host platform.
final BinaryMessenger? binaryMessenger;
/// Maintains instances stored to communicate with native language objects.
final InstanceManager instanceManager;
@override
void create(int identifier) {
instanceManager.addHostCreatedInstance(
PendingRecording.detached(
binaryMessenger: binaryMessenger,
instanceManager: instanceManager,
),
identifier, onCopy: (PendingRecording original) {
return PendingRecording.detached(
binaryMessenger: binaryMessenger,
instanceManager: instanceManager,
);
});
}
}
| packages/packages/camera/camera_android_camerax/lib/src/pending_recording.dart/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/lib/src/pending_recording.dart",
"repo_id": "packages",
"token_count": 1159
} | 943 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:camera_android_camerax/src/analyzer.dart';
import 'package:camera_android_camerax/src/image_proxy.dart';
import 'package:camera_android_camerax/src/instance_manager.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:mockito/annotations.dart';
import 'package:mockito/mockito.dart';
import 'analyzer_test.mocks.dart';
import 'test_camerax_library.g.dart';
@GenerateMocks(<Type>[TestAnalyzerHostApi, TestInstanceManagerHostApi])
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
// Mocks the call to clear the native InstanceManager.
TestInstanceManagerHostApi.setup(MockTestInstanceManagerHostApi());
group('Analyzer', () {
setUp(() {});
tearDown(() {
TestAnalyzerHostApi.setup(null);
});
test('HostApi create', () {
final MockTestAnalyzerHostApi mockApi = MockTestAnalyzerHostApi();
TestAnalyzerHostApi.setup(mockApi);
final InstanceManager instanceManager = InstanceManager(
onWeakReferenceRemoved: (_) {},
);
final Analyzer instance = Analyzer(
analyze: (ImageProxy imageProxy) async {},
instanceManager: instanceManager,
);
verify(mockApi.create(
instanceManager.getIdentifier(instance),
));
});
test('FlutterAPI create', () {
final InstanceManager instanceManager = InstanceManager(
onWeakReferenceRemoved: (_) {},
);
final AnalyzerFlutterApiImpl api = AnalyzerFlutterApiImpl(
instanceManager: instanceManager,
);
const int instanceIdentifier = 0;
api.create(
instanceIdentifier,
);
expect(
instanceManager.getInstanceWithWeakReference(instanceIdentifier),
isA<Analyzer>(),
);
});
test('analyze', () {
final InstanceManager instanceManager = InstanceManager(
onWeakReferenceRemoved: (_) {},
);
const int instanceIdentifier = 0;
const int imageProxyIdentifier = 44;
late final Object callbackParameter;
final Analyzer instance = Analyzer.detached(
analyze: (
ImageProxy imageProxy,
) async {
callbackParameter = imageProxy;
},
instanceManager: instanceManager,
);
instanceManager.addHostCreatedInstance(
instance,
instanceIdentifier,
onCopy: (Analyzer original) => Analyzer.detached(
analyze: original.analyze,
instanceManager: instanceManager,
),
);
final ImageProxy imageProxy = ImageProxy.detached(
instanceManager: instanceManager, format: 3, height: 4, width: 5);
instanceManager.addHostCreatedInstance(imageProxy, imageProxyIdentifier,
onCopy: (ImageProxy original) => ImageProxy.detached(
instanceManager: instanceManager,
format: original.format,
height: original.height,
width: original.width));
final AnalyzerFlutterApiImpl flutterApi = AnalyzerFlutterApiImpl(
instanceManager: instanceManager,
);
flutterApi.analyze(
instanceIdentifier,
imageProxyIdentifier,
);
expect(
callbackParameter,
imageProxy,
);
});
});
}
| packages/packages/camera/camera_android_camerax/test/analyzer_test.dart/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/test/analyzer_test.dart",
"repo_id": "packages",
"token_count": 1328
} | 944 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:camera_android_camerax/src/camera_state.dart';
import 'package:camera_android_camerax/src/camera_state_error.dart';
import 'package:camera_android_camerax/src/camerax_library.g.dart';
import 'package:camera_android_camerax/src/instance_manager.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:mockito/annotations.dart';
import 'camera_state_test.mocks.dart';
import 'test_camerax_library.g.dart';
@GenerateMocks(<Type>[TestInstanceManagerHostApi])
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
// Mocks the call to clear the native InstanceManager.
TestInstanceManagerHostApi.setup(MockTestInstanceManagerHostApi());
group('CameraState', () {
test(
'FlutterAPI create makes call to create CameraState instance with expected identifier',
() {
final InstanceManager instanceManager = InstanceManager(
onWeakReferenceRemoved: (_) {},
);
final CameraStateFlutterApiImpl api = CameraStateFlutterApiImpl(
instanceManager: instanceManager,
);
// Create CameraStateError for CameraState instance.
const int code = 23;
final CameraStateError cameraStateError = CameraStateError.detached(
instanceManager: instanceManager,
code: code,
);
final int cameraStateErrorIdentifier =
instanceManager.addDartCreatedInstance(cameraStateError, onCopy: (_) {
return CameraStateError.detached(code: code);
});
// Create CameraState.
const int instanceIdentifier = 46;
const CameraStateType cameraStateType = CameraStateType.closed;
api.create(
instanceIdentifier,
CameraStateTypeData(value: cameraStateType),
cameraStateErrorIdentifier,
);
// Test instance type.
final Object? instance =
instanceManager.getInstanceWithWeakReference(instanceIdentifier);
expect(
instanceManager.getInstanceWithWeakReference(instanceIdentifier),
isA<CameraState>(),
);
// Test instance properties.
final CameraState cameraState = instance! as CameraState;
expect(cameraState.type, equals(cameraStateType));
expect(cameraState.error, equals(cameraStateError));
});
});
}
| packages/packages/camera/camera_android_camerax/test/camera_state_test.dart/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/test/camera_state_test.dart",
"repo_id": "packages",
"token_count": 841
} | 945 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:camera_android_camerax/src/analyzer.dart';
import 'package:camera_android_camerax/src/image_analysis.dart';
import 'package:camera_android_camerax/src/image_proxy.dart';
import 'package:camera_android_camerax/src/instance_manager.dart';
import 'package:camera_android_camerax/src/resolution_selector.dart';
import 'package:camera_android_camerax/src/surface.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:mockito/annotations.dart';
import 'package:mockito/mockito.dart';
import 'image_analysis_test.mocks.dart';
import 'test_camerax_library.g.dart';
@GenerateMocks(<Type>[
TestImageAnalysisHostApi,
TestInstanceManagerHostApi,
ResolutionSelector,
])
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
// Mocks the call to clear the native InstanceManager.
TestInstanceManagerHostApi.setup(MockTestInstanceManagerHostApi());
group('ImageAnalysis', () {
tearDown(() {
TestImageAnalysisHostApi.setup(null);
});
test('detached create does not call create on the Java side', () {
final MockTestImageAnalysisHostApi mockApi =
MockTestImageAnalysisHostApi();
TestImageAnalysisHostApi.setup(mockApi);
final InstanceManager instanceManager = InstanceManager(
onWeakReferenceRemoved: (_) {},
);
ImageAnalysis.detached(
initialTargetRotation: Surface.ROTATION_270,
resolutionSelector: MockResolutionSelector(),
instanceManager: instanceManager,
);
verifyNever(mockApi.create(argThat(isA<int>()), argThat(isA<int>()),
argThat(isA<ResolutionSelector>())));
});
test('create calls create on the Java side', () {
final MockTestImageAnalysisHostApi mockApi =
MockTestImageAnalysisHostApi();
TestImageAnalysisHostApi.setup(mockApi);
final InstanceManager instanceManager = InstanceManager(
onWeakReferenceRemoved: (_) {},
);
const int targetRotation = Surface.ROTATION_90;
final MockResolutionSelector mockResolutionSelector =
MockResolutionSelector();
const int mockResolutionSelectorId = 24;
instanceManager.addHostCreatedInstance(
mockResolutionSelector, mockResolutionSelectorId,
onCopy: (ResolutionSelector original) {
return MockResolutionSelector();
});
final ImageAnalysis instance = ImageAnalysis(
initialTargetRotation: targetRotation,
resolutionSelector: mockResolutionSelector,
instanceManager: instanceManager,
);
verify(mockApi.create(
argThat(equals(instanceManager.getIdentifier(instance))),
argThat(equals(targetRotation)),
argThat(equals(mockResolutionSelectorId))));
});
test(
'setTargetRotation makes call to set target rotation for ImageAnalysis instance',
() async {
final MockTestImageAnalysisHostApi mockApi =
MockTestImageAnalysisHostApi();
TestImageAnalysisHostApi.setup(mockApi);
final InstanceManager instanceManager = InstanceManager(
onWeakReferenceRemoved: (_) {},
);
const int targetRotation = Surface.ROTATION_180;
final ImageAnalysis imageAnalysis = ImageAnalysis.detached(
instanceManager: instanceManager,
);
instanceManager.addHostCreatedInstance(
imageAnalysis,
0,
onCopy: (_) => ImageAnalysis.detached(instanceManager: instanceManager),
);
await imageAnalysis.setTargetRotation(targetRotation);
verify(mockApi.setTargetRotation(
instanceManager.getIdentifier(imageAnalysis), targetRotation));
});
test('setAnalyzer makes call to set analyzer on ImageAnalysis instance',
() async {
final MockTestImageAnalysisHostApi mockApi =
MockTestImageAnalysisHostApi();
TestImageAnalysisHostApi.setup(mockApi);
final InstanceManager instanceManager = InstanceManager(
onWeakReferenceRemoved: (_) {},
);
final ImageAnalysis instance = ImageAnalysis.detached(
resolutionSelector: MockResolutionSelector(),
instanceManager: instanceManager,
);
const int instanceIdentifier = 0;
instanceManager.addHostCreatedInstance(
instance,
instanceIdentifier,
onCopy: (ImageAnalysis original) => ImageAnalysis.detached(
resolutionSelector: original.resolutionSelector,
instanceManager: instanceManager,
),
);
final Analyzer analyzer = Analyzer.detached(
analyze: (ImageProxy imageProxy) async {},
instanceManager: instanceManager,
);
const int analyzerIdentifier = 10;
instanceManager.addHostCreatedInstance(
analyzer,
analyzerIdentifier,
onCopy: (_) => Analyzer.detached(
analyze: (ImageProxy imageProxy) async {},
instanceManager: instanceManager,
),
);
await instance.setAnalyzer(
analyzer,
);
verify(mockApi.setAnalyzer(
instanceIdentifier,
analyzerIdentifier,
));
});
test('clearAnalyzer makes call to clear analyzer on ImageAnalysis instance',
() async {
final MockTestImageAnalysisHostApi mockApi =
MockTestImageAnalysisHostApi();
TestImageAnalysisHostApi.setup(mockApi);
final InstanceManager instanceManager = InstanceManager(
onWeakReferenceRemoved: (_) {},
);
final ImageAnalysis instance = ImageAnalysis.detached(
resolutionSelector: MockResolutionSelector(),
instanceManager: instanceManager,
);
const int instanceIdentifier = 0;
instanceManager.addHostCreatedInstance(
instance,
instanceIdentifier,
onCopy: (ImageAnalysis original) => ImageAnalysis.detached(
resolutionSelector: original.resolutionSelector,
instanceManager: instanceManager,
),
);
await instance.clearAnalyzer();
verify(mockApi.clearAnalyzer(
instanceIdentifier,
));
});
});
}
| packages/packages/camera/camera_android_camerax/test/image_analysis_test.dart/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/test/image_analysis_test.dart",
"repo_id": "packages",
"token_count": 2341
} | 946 |
// Mocks generated by Mockito 5.4.4 from annotations
// in camera_android_camerax/test/system_services_test.dart.
// Do not manually edit this file.
// ignore_for_file: no_leading_underscores_for_library_prefixes
import 'dart:async' as _i3;
import 'package:camera_android_camerax/src/camerax_library.g.dart' as _i4;
import 'package:mockito/mockito.dart' as _i1;
import 'package:mockito/src/dummies.dart' as _i5;
import 'test_camerax_library.g.dart' as _i2;
// ignore_for_file: type=lint
// ignore_for_file: avoid_redundant_argument_values
// ignore_for_file: avoid_setters_without_getters
// ignore_for_file: comment_references
// ignore_for_file: deprecated_member_use
// ignore_for_file: deprecated_member_use_from_same_package
// ignore_for_file: implementation_imports
// ignore_for_file: invalid_use_of_visible_for_testing_member
// ignore_for_file: prefer_const_constructors
// ignore_for_file: unnecessary_parenthesis
// ignore_for_file: camel_case_types
// ignore_for_file: subtype_of_sealed_class
/// A class which mocks [TestInstanceManagerHostApi].
///
/// See the documentation for Mockito's code generation for more information.
class MockTestInstanceManagerHostApi extends _i1.Mock
implements _i2.TestInstanceManagerHostApi {
MockTestInstanceManagerHostApi() {
_i1.throwOnMissingStub(this);
}
@override
void clear() => super.noSuchMethod(
Invocation.method(
#clear,
[],
),
returnValueForMissingStub: null,
);
}
/// A class which mocks [TestSystemServicesHostApi].
///
/// See the documentation for Mockito's code generation for more information.
class MockTestSystemServicesHostApi extends _i1.Mock
implements _i2.TestSystemServicesHostApi {
MockTestSystemServicesHostApi() {
_i1.throwOnMissingStub(this);
}
@override
_i3.Future<_i4.CameraPermissionsErrorData?> requestCameraPermissions(
bool? enableAudio) =>
(super.noSuchMethod(
Invocation.method(
#requestCameraPermissions,
[enableAudio],
),
returnValue: _i3.Future<_i4.CameraPermissionsErrorData?>.value(),
) as _i3.Future<_i4.CameraPermissionsErrorData?>);
@override
String getTempFilePath(
String? prefix,
String? suffix,
) =>
(super.noSuchMethod(
Invocation.method(
#getTempFilePath,
[
prefix,
suffix,
],
),
returnValue: _i5.dummyValue<String>(
this,
Invocation.method(
#getTempFilePath,
[
prefix,
suffix,
],
),
),
) as String);
}
| packages/packages/camera/camera_android_camerax/test/system_services_test.mocks.dart/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/test/system_services_test.mocks.dart",
"repo_id": "packages",
"token_count": 1096
} | 947 |
## NEXT
* Updates minimum supported SDK version to Flutter 3.13/Dart 3.1.
## 0.2.1+9
* Updates minimum supported SDK version to Flutter 3.10/Dart 3.0.
* Fixes new lint warnings.
## 0.2.1+8
* Adds pub topics to package metadata.
* Updates minimum supported SDK version to Flutter 3.7/Dart 2.19.
## 0.2.1+7
* Fixes unawaited_futures violations.
* Updates minimum supported SDK version to Flutter 3.3/Dart 2.18.
## 0.2.1+6
* Sets a cmake_policy compatibility version to fix build warnings.
* Aligns Dart and Flutter SDK constraints.
## 0.2.1+5
* Updates links for the merge of flutter/plugins into flutter/packages.
* Updates minimum Flutter version to 3.0.
## 0.2.1+4
* Updates code for stricter lint checks.
## 0.2.1+3
* Updates to latest camera platform interface but fails if user attempts to use streaming with recording (since streaming is currently unsupported on Windows).
## 0.2.1+2
* Updates code for `no_leading_underscores_for_local_identifiers` lint.
* Updates minimum Flutter version to 2.10.
## 0.2.1+1
* Fixes avoid_redundant_argument_values lint warnings and minor typos.
## 0.2.1
* Adds a check for string size before Win32 MultiByte <-> WideChar conversions
## 0.2.0
**BREAKING CHANGES**:
* `CameraException.code` now has value `"CameraAccessDenied"` if camera access permission was denied.
* `CameraException.code` now has value `"camera_error"` if error occurs during capture.
## 0.1.0+5
* Fixes bugs in in error handling.
## 0.1.0+4
* Allows retrying camera initialization after error.
## 0.1.0+3
* Updates the README to better explain how to use the unendorsed package.
## 0.1.0+2
* Updates references to the obsolete master branch.
## 0.1.0+1
* Removes unnecessary imports.
* Fixes library_private_types_in_public_api, sort_child_properties_last and use_key_in_widget_constructors
lint warnings.
## 0.1.0
* Initial release
| packages/packages/camera/camera_windows/CHANGELOG.md/0 | {
"file_path": "packages/packages/camera/camera_windows/CHANGELOG.md",
"repo_id": "packages",
"token_count": 620
} | 948 |
# An example app for staggered & wrap layout
| packages/packages/dynamic_layouts/example/README.md/0 | {
"file_path": "packages/packages/dynamic_layouts/example/README.md",
"repo_id": "packages",
"token_count": 10
} | 949 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:example/wrap_layout_example.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Check wrap layout', (WidgetTester tester) async {
const MaterialApp app = MaterialApp(
home: WrapExample(),
);
await tester.pumpWidget(app);
await tester.pumpAndSettle();
// Validate which children are laid out.
for (int i = 0; i <= 12; i++) {
expect(find.text('Index $i'), findsOneWidget);
}
for (int i = 13; i < 19; i++) {
expect(find.text('Index $i'), findsNothing);
}
// Validate with the position of the box, not the text.
Finder getContainer(String text) {
return find.ancestor(
of: find.text(text),
matching: find.byType(Container),
);
}
// Validate layout position.
expect(
tester.getTopLeft(getContainer('Index 0')),
const Offset(0.0, 56.0),
);
expect(
tester.getTopLeft(getContainer('Index 1')),
const Offset(40.0, 56.0),
);
expect(
tester.getTopLeft(getContainer('Index 2')),
const Offset(190.0, 56.0),
);
expect(
tester.getTopLeft(getContainer('Index 3')),
const Offset(270.0, 56.0),
);
expect(
tester.getTopLeft(getContainer('Index 4')),
const Offset(370.0, 56.0),
);
expect(
tester.getTopLeft(getContainer('Index 5')),
const Offset(490.0, 56.0),
);
expect(
tester.getTopLeft(getContainer('Index 6')),
const Offset(690.0, 56.0),
);
expect(
tester.getTopLeft(getContainer('Index 7')),
const Offset(0.0, 506.0),
);
expect(
tester.getTopLeft(getContainer('Index 8')),
const Offset(150.0, 506.0),
);
expect(
tester.getTopLeft(getContainer('Index 9')),
const Offset(250.0, 506.0),
);
expect(
tester.getTopLeft(getContainer('Index 10')),
const Offset(350.0, 506.0),
);
expect(
tester.getTopLeft(getContainer('Index 11')),
const Offset(390.0, 506.0),
);
expect(
tester.getTopLeft(getContainer('Index 12')),
const Offset(590.0, 506.0),
);
});
}
| packages/packages/dynamic_layouts/example/test/wrap_example_test.dart/0 | {
"file_path": "packages/packages/dynamic_layouts/example/test/wrap_example_test.dart",
"repo_id": "packages",
"token_count": 1015
} | 950 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:dynamic_layouts/dynamic_layouts.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('DynamicGridView works with simple layout',
(WidgetTester tester) async {
// Can have no children
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: DynamicGridView(
gridDelegate: TestDelegate(crossAxisCount: 2),
),
),
),
);
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: DynamicGridView(
gridDelegate: TestDelegate(crossAxisCount: 2),
children: List<Widget>.generate(
50,
(int index) => SizedBox.square(
dimension: TestSimpleLayout.childExtent,
child: Text('Index $index'),
),
),
),
),
),
);
// Only the visible tiles have been laid out.
expect(find.text('Index 0'), findsOneWidget);
expect(tester.getTopLeft(find.text('Index 0')), Offset.zero);
expect(find.text('Index 1'), findsOneWidget);
expect(tester.getTopLeft(find.text('Index 1')), const Offset(50.0, 0.0));
expect(find.text('Index 2'), findsOneWidget);
expect(tester.getTopLeft(find.text('Index 2')), const Offset(0.0, 50.0));
expect(find.text('Index 47'), findsNothing);
expect(find.text('Index 48'), findsNothing);
expect(find.text('Index 49'), findsNothing);
});
testWidgets('DynamicGridView.builder works with simple layout',
(WidgetTester tester) async {
// Only a few number of tiles
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: DynamicGridView.builder(
gridDelegate: TestDelegate(crossAxisCount: 2),
itemCount: 3,
itemBuilder: (BuildContext context, int index) {
return SizedBox.square(
dimension: TestSimpleLayout.childExtent,
child: Text('Index $index'),
);
},
),
),
),
);
// Only the visible tiles have been laid out, up to itemCount.
expect(find.text('Index 0'), findsOneWidget);
expect(tester.getTopLeft(find.text('Index 0')), Offset.zero);
expect(find.text('Index 1'), findsOneWidget);
expect(tester.getTopLeft(find.text('Index 1')), const Offset(50.0, 0.0));
expect(find.text('Index 2'), findsOneWidget);
expect(tester.getTopLeft(find.text('Index 2')), const Offset(0.0, 50.0));
expect(find.text('Index 3'), findsNothing);
expect(find.text('Index 4'), findsNothing);
expect(find.text('Index 5'), findsNothing);
// Infinite number of tiles
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: DynamicGridView.builder(
gridDelegate: TestDelegate(crossAxisCount: 2),
itemBuilder: (BuildContext context, int index) {
return SizedBox.square(
dimension: TestSimpleLayout.childExtent,
child: Text('Index $index'),
);
},
),
),
),
);
// Only the visible tiles have been laid out.
expect(find.text('Index 0'), findsOneWidget);
expect(tester.getTopLeft(find.text('Index 0')), Offset.zero);
expect(find.text('Index 1'), findsOneWidget);
expect(tester.getTopLeft(find.text('Index 1')), const Offset(50.0, 0.0));
expect(find.text('Index 2'), findsOneWidget);
expect(tester.getTopLeft(find.text('Index 2')), const Offset(0.0, 50.0));
expect(find.text('Index 47'), findsNothing);
expect(find.text('Index 48'), findsNothing);
expect(find.text('Index 49'), findsNothing);
});
}
class TestSimpleLayout extends DynamicSliverGridLayout {
TestSimpleLayout({
required this.crossAxisCount,
});
final int crossAxisCount;
static const double childExtent = 50.0;
@override
DynamicSliverGridGeometry getGeometryForChildIndex(int index) {
final double crossAxisStart = (index % crossAxisCount) * childExtent;
return DynamicSliverGridGeometry(
scrollOffset: (index ~/ crossAxisCount) * childExtent,
crossAxisOffset: crossAxisStart,
mainAxisExtent: childExtent,
crossAxisExtent: childExtent,
);
}
@override
bool reachedTargetScrollOffset(double targetOffset) => true;
@override
DynamicSliverGridGeometry updateGeometryForChildIndex(
int index,
Size childSize,
) {
return getGeometryForChildIndex(index);
}
}
class TestDelegate extends SliverGridDelegateWithFixedCrossAxisCount {
TestDelegate({required super.crossAxisCount});
@override
DynamicSliverGridLayout getLayout(SliverConstraints constraints) {
return TestSimpleLayout(crossAxisCount: crossAxisCount);
}
}
| packages/packages/dynamic_layouts/test/dynamic_grid_test.dart/0 | {
"file_path": "packages/packages/dynamic_layouts/test/dynamic_grid_test.dart",
"repo_id": "packages",
"token_count": 2031
} | 951 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:google_sign_in/google_sign_in.dart';
import 'package:googleapis_auth/googleapis_auth.dart' as gapis;
import 'package:http/http.dart' as http;
import 'package:meta/meta.dart';
/// Extension on [GoogleSignIn] that adds an `authenticatedClient` method.
///
/// This method can be used to retrieve an authenticated [gapis.AuthClient]
/// client that can be used with the rest of the `googleapis` libraries.
extension GoogleApisGoogleSignInAuth on GoogleSignIn {
/// Retrieve a `googleapis` authenticated client.
Future<gapis.AuthClient?> authenticatedClient({
@visibleForTesting GoogleSignInAuthentication? debugAuthentication,
@visibleForTesting List<String>? debugScopes,
}) async {
final GoogleSignInAuthentication? auth =
debugAuthentication ?? await currentUser?.authentication;
final String? oauthTokenString = auth?.accessToken;
if (oauthTokenString == null) {
return null;
}
final gapis.AccessCredentials credentials = gapis.AccessCredentials(
gapis.AccessToken(
'Bearer',
oauthTokenString,
// TODO(kevmoo): Use the correct value once it's available from authentication
// See https://github.com/flutter/flutter/issues/80905
DateTime.now().toUtc().add(const Duration(days: 365)),
),
null, // We don't have a refreshToken
debugScopes ?? scopes,
);
return gapis.authenticatedClient(http.Client(), credentials);
}
}
| packages/packages/extension_google_sign_in_as_googleapis_auth/lib/extension_google_sign_in_as_googleapis_auth.dart/0 | {
"file_path": "packages/packages/extension_google_sign_in_as_googleapis_auth/lib/extension_google_sign_in_as_googleapis_auth.dart",
"repo_id": "packages",
"token_count": 535
} | 952 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package dev.flutter.packages.file_selector_android;
import android.app.Activity;
import android.content.ClipData;
import android.content.ContentResolver;
import android.content.Intent;
import android.database.Cursor;
import android.net.Uri;
import android.os.Build;
import android.provider.DocumentsContract;
import android.provider.OpenableColumns;
import android.util.Log;
import android.webkit.MimeTypeMap;
import androidx.annotation.ChecksSdkIntAtLeast;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.annotation.VisibleForTesting;
import io.flutter.embedding.engine.plugins.activity.ActivityPluginBinding;
import io.flutter.plugin.common.PluginRegistry;
import java.io.DataInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class FileSelectorApiImpl implements GeneratedFileSelectorApi.FileSelectorApi {
private static final String TAG = "FileSelectorApiImpl";
// Request code for selecting a file.
private static final int OPEN_FILE = 221;
// Request code for selecting files.
private static final int OPEN_FILES = 222;
// Request code for selecting a directory.
private static final int OPEN_DIR = 223;
private final @NonNull NativeObjectFactory objectFactory;
private final @NonNull AndroidSdkChecker sdkChecker;
@Nullable ActivityPluginBinding activityPluginBinding;
private abstract static class OnResultListener {
public abstract void onResult(int resultCode, @Nullable Intent data);
}
// Handles instantiating class objects that are needed by this class. This is provided to be
// overridden for tests.
@VisibleForTesting
static class NativeObjectFactory {
@NonNull
Intent newIntent(@NonNull String action) {
return new Intent(action);
}
@NonNull
DataInputStream newDataInputStream(InputStream inputStream) {
return new DataInputStream(inputStream);
}
}
// Interface for an injectable SDK version checker.
@VisibleForTesting
interface AndroidSdkChecker {
@ChecksSdkIntAtLeast(parameter = 0)
boolean sdkIsAtLeast(int version);
}
public FileSelectorApiImpl(@NonNull ActivityPluginBinding activityPluginBinding) {
this(
activityPluginBinding,
new NativeObjectFactory(),
(int version) -> Build.VERSION.SDK_INT >= version);
}
@VisibleForTesting
FileSelectorApiImpl(
@NonNull ActivityPluginBinding activityPluginBinding,
@NonNull NativeObjectFactory objectFactory,
@NonNull AndroidSdkChecker sdkChecker) {
this.activityPluginBinding = activityPluginBinding;
this.objectFactory = objectFactory;
this.sdkChecker = sdkChecker;
}
@Override
public void openFile(
@Nullable String initialDirectory,
@NonNull GeneratedFileSelectorApi.FileTypes allowedTypes,
@NonNull GeneratedFileSelectorApi.Result<GeneratedFileSelectorApi.FileResponse> result) {
final Intent intent = objectFactory.newIntent(Intent.ACTION_OPEN_DOCUMENT);
intent.addCategory(Intent.CATEGORY_OPENABLE);
setMimeTypes(intent, allowedTypes);
trySetInitialDirectory(intent, initialDirectory);
try {
startActivityForResult(
intent,
OPEN_FILE,
new OnResultListener() {
@Override
public void onResult(int resultCode, @Nullable Intent data) {
if (resultCode == Activity.RESULT_OK && data != null) {
final Uri uri = data.getData();
final GeneratedFileSelectorApi.FileResponse file = toFileResponse(uri);
if (file != null) {
result.success(file);
} else {
result.error(new Exception("Failed to read file: " + uri));
}
} else {
result.success(null);
}
}
});
} catch (Exception exception) {
result.error(exception);
}
}
@Override
public void openFiles(
@Nullable String initialDirectory,
@NonNull GeneratedFileSelectorApi.FileTypes allowedTypes,
@NonNull
GeneratedFileSelectorApi.Result<List<GeneratedFileSelectorApi.FileResponse>> result) {
final Intent intent = objectFactory.newIntent(Intent.ACTION_OPEN_DOCUMENT);
intent.addCategory(Intent.CATEGORY_OPENABLE);
intent.putExtra(Intent.EXTRA_ALLOW_MULTIPLE, true);
setMimeTypes(intent, allowedTypes);
trySetInitialDirectory(intent, initialDirectory);
try {
startActivityForResult(
intent,
OPEN_FILES,
new OnResultListener() {
@Override
public void onResult(int resultCode, @Nullable Intent data) {
if (resultCode == Activity.RESULT_OK && data != null) {
// Only one file was returned.
final Uri uri = data.getData();
if (uri != null) {
final GeneratedFileSelectorApi.FileResponse file = toFileResponse(uri);
if (file != null) {
result.success(Collections.singletonList(file));
} else {
result.error(new Exception("Failed to read file: " + uri));
}
}
// Multiple files were returned.
final ClipData clipData = data.getClipData();
if (clipData != null) {
final List<GeneratedFileSelectorApi.FileResponse> files =
new ArrayList<>(clipData.getItemCount());
for (int i = 0; i < clipData.getItemCount(); i++) {
final ClipData.Item clipItem = clipData.getItemAt(i);
final GeneratedFileSelectorApi.FileResponse file =
toFileResponse(clipItem.getUri());
if (file != null) {
files.add(file);
} else {
result.error(new Exception("Failed to read file: " + uri));
return;
}
}
result.success(files);
}
} else {
result.success(new ArrayList<>());
}
}
});
} catch (Exception exception) {
result.error(exception);
}
}
@Override
public void getDirectoryPath(
@Nullable String initialDirectory, @NonNull GeneratedFileSelectorApi.Result<String> result) {
if (!sdkChecker.sdkIsAtLeast(android.os.Build.VERSION_CODES.LOLLIPOP)) {
result.error(
new UnsupportedOperationException(
"Selecting a directory is only supported on versions >= 21"));
return;
}
final Intent intent = objectFactory.newIntent(Intent.ACTION_OPEN_DOCUMENT_TREE);
trySetInitialDirectory(intent, initialDirectory);
try {
startActivityForResult(
intent,
OPEN_DIR,
new OnResultListener() {
@Override
public void onResult(int resultCode, @Nullable Intent data) {
if (resultCode == Activity.RESULT_OK && data != null) {
final Uri uri = data.getData();
result.success(uri.toString());
} else {
result.success(null);
}
}
});
} catch (Exception exception) {
result.error(exception);
}
}
public void setActivityPluginBinding(@Nullable ActivityPluginBinding activityPluginBinding) {
this.activityPluginBinding = activityPluginBinding;
}
// Setting the mimeType with `setType` is required when opening files. This handles setting the
// mimeType based on the `mimeTypes` list and converts extensions to mimeTypes.
// See https://developer.android.com/guide/components/intents-common#OpenFile
private void setMimeTypes(
@NonNull Intent intent, @NonNull GeneratedFileSelectorApi.FileTypes allowedTypes) {
final Set<String> allMimetypes = new HashSet<>();
allMimetypes.addAll(allowedTypes.getMimeTypes());
allMimetypes.addAll(tryConvertExtensionsToMimetypes(allowedTypes.getExtensions()));
if (allMimetypes.isEmpty()) {
intent.setType("*/*");
} else if (allMimetypes.size() == 1) {
intent.setType(allMimetypes.iterator().next());
} else {
intent.setType("*/*");
intent.putExtra(Intent.EXTRA_MIME_TYPES, allMimetypes.toArray(new String[0]));
}
}
// Attempts to convert each extension to Android compatible mimeType. Logs a warning if an
// extension could not be converted.
@NonNull
private List<String> tryConvertExtensionsToMimetypes(@NonNull List<String> extensions) {
if (extensions.isEmpty()) {
return Collections.emptyList();
}
final MimeTypeMap mimeTypeMap = MimeTypeMap.getSingleton();
final Set<String> mimeTypes = new HashSet<>();
for (String extension : extensions) {
final String mimetype = mimeTypeMap.getMimeTypeFromExtension(extension);
if (mimetype != null) {
mimeTypes.add(mimetype);
} else {
Log.w(TAG, "Extension not supported: " + extension);
}
}
return new ArrayList<>(mimeTypes);
}
private void trySetInitialDirectory(@NonNull Intent intent, @Nullable String initialDirectory) {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O && initialDirectory != null) {
intent.putExtra(DocumentsContract.EXTRA_INITIAL_URI, Uri.parse(initialDirectory));
}
}
private void startActivityForResult(
@NonNull Intent intent, int attemptRequestCode, @NonNull OnResultListener resultListener)
throws Exception {
if (activityPluginBinding == null) {
throw new Exception("No activity is available.");
}
activityPluginBinding.addActivityResultListener(
new PluginRegistry.ActivityResultListener() {
@Override
public boolean onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
if (requestCode == attemptRequestCode) {
resultListener.onResult(resultCode, data);
activityPluginBinding.removeActivityResultListener(this);
return true;
}
return false;
}
});
activityPluginBinding.getActivity().startActivityForResult(intent, attemptRequestCode);
}
@Nullable
GeneratedFileSelectorApi.FileResponse toFileResponse(@NonNull Uri uri) {
if (activityPluginBinding == null) {
Log.d(TAG, "Activity is not available.");
return null;
}
final ContentResolver contentResolver =
activityPluginBinding.getActivity().getContentResolver();
String name = null;
Integer size = null;
try (Cursor cursor = contentResolver.query(uri, null, null, null, null, null)) {
if (cursor != null && cursor.moveToFirst()) {
// Note it's called "Display Name". This is
// provider-specific, and might not necessarily be the file name.
final int nameIndex = cursor.getColumnIndex(OpenableColumns.DISPLAY_NAME);
if (nameIndex >= 0) {
name = cursor.getString(nameIndex);
}
final int sizeIndex = cursor.getColumnIndex(OpenableColumns.SIZE);
// If the size is unknown, the value stored is null. This will
// happen often: The storage API allows for remote files, whose
// size might not be locally known.
if (!cursor.isNull(sizeIndex)) {
size = cursor.getInt(sizeIndex);
}
}
}
if (size == null) {
return null;
}
final byte[] bytes = new byte[size];
try (InputStream inputStream = contentResolver.openInputStream(uri)) {
final DataInputStream dataInputStream = objectFactory.newDataInputStream(inputStream);
dataInputStream.readFully(bytes);
} catch (IOException exception) {
Log.w(TAG, exception.getMessage());
return null;
}
return new GeneratedFileSelectorApi.FileResponse.Builder()
.setName(name)
.setBytes(bytes)
.setPath(uri.toString())
.setMimeType(contentResolver.getType(uri))
.setSize(size.longValue())
.build();
}
}
| packages/packages/file_selector/file_selector_android/android/src/main/java/dev/flutter/packages/file_selector_android/FileSelectorApiImpl.java/0 | {
"file_path": "packages/packages/file_selector/file_selector_android/android/src/main/java/dev/flutter/packages/file_selector_android/FileSelectorApiImpl.java",
"repo_id": "packages",
"token_count": 4921
} | 953 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:file_selector_platform_interface/file_selector_platform_interface.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
/// Screen that allows the user to select multiple image files using
/// `openFiles`, then displays the selected images in a gallery dialog.
class OpenMultipleImagesPage extends StatelessWidget {
/// Default Constructor
const OpenMultipleImagesPage({super.key});
Future<void> _openImageFile(BuildContext context) async {
const XTypeGroup jpgsTypeGroup = XTypeGroup(
label: 'JPEGs',
extensions: <String>['jpg', 'jpeg'],
uniformTypeIdentifiers: <String>['public.jpeg'],
);
const XTypeGroup pngTypeGroup = XTypeGroup(
label: 'PNGs',
extensions: <String>['png'],
uniformTypeIdentifiers: <String>['public.png'],
);
final List<XFile> files = await FileSelectorPlatform.instance
.openFiles(acceptedTypeGroups: <XTypeGroup>[
jpgsTypeGroup,
pngTypeGroup,
]);
if (files.isEmpty) {
// Operation was canceled by the user.
return;
}
final List<Uint8List> imageBytes = <Uint8List>[];
for (final XFile file in files) {
imageBytes.add(await file.readAsBytes());
}
if (context.mounted) {
await showDialog<void>(
context: context,
builder: (BuildContext context) => MultipleImagesDisplay(imageBytes),
);
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Open multiple images'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
style: ElevatedButton.styleFrom(
foregroundColor: Colors.blue,
backgroundColor: Colors.white,
),
child: const Text('Press to open multiple images (png, jpg)'),
onPressed: () => _openImageFile(context),
),
],
),
),
);
}
}
/// Widget that displays a text file in a dialog.
class MultipleImagesDisplay extends StatelessWidget {
/// Default Constructor.
const MultipleImagesDisplay(this.fileBytes, {super.key});
/// The bytes containing the images.
final List<Uint8List> fileBytes;
@override
Widget build(BuildContext context) {
return AlertDialog(
title: const Text('Gallery'),
// On web the filePath is a blob url
// while on other platforms it is a system path.
content: Center(
child: Row(
children: <Widget>[
for (int i = 0; i < fileBytes.length; i++)
Flexible(
key: Key('result_image_name$i'),
child: Image.memory(fileBytes[i]),
)
],
),
),
actions: <Widget>[
TextButton(
child: const Text('Close'),
onPressed: () {
Navigator.pop(context);
},
),
],
);
}
}
| packages/packages/file_selector/file_selector_android/example/lib/open_multiple_images_page.dart/0 | {
"file_path": "packages/packages/file_selector/file_selector_android/example/lib/open_multiple_images_page.dart",
"repo_id": "packages",
"token_count": 1319
} | 954 |
#include "../../Flutter/Flutter-Release.xcconfig"
#include "Warnings.xcconfig"
| packages/packages/file_selector/file_selector_macos/example/macos/Runner/Configs/Release.xcconfig/0 | {
"file_path": "packages/packages/file_selector/file_selector_macos/example/macos/Runner/Configs/Release.xcconfig",
"repo_id": "packages",
"token_count": 32
} | 955 |
name: file_selector_macos
description: macOS implementation of the file_selector plugin.
repository: https://github.com/flutter/packages/tree/main/packages/file_selector/file_selector_macos
issue_tracker: https://github.com/flutter/flutter/issues?q=is%3Aissue+is%3Aopen+label%3A%22p%3A+file_selector%22
version: 0.9.3+3
environment:
sdk: ^3.1.0
flutter: ">=3.13.0"
flutter:
plugin:
implements: file_selector
platforms:
macos:
dartPluginClass: FileSelectorMacOS
pluginClass: FileSelectorPlugin
dependencies:
cross_file: ^0.3.1
file_selector_platform_interface: ^2.6.0
flutter:
sdk: flutter
dev_dependencies:
build_runner: ^2.3.2
flutter_test:
sdk: flutter
mockito: 5.4.4
pigeon: ^10.1.3
topics:
- files
- file-selection
- file-selector
| packages/packages/file_selector/file_selector_macos/pubspec.yaml/0 | {
"file_path": "packages/packages/file_selector/file_selector_macos/pubspec.yaml",
"repo_id": "packages",
"token_count": 338
} | 956 |
name: file_selector_platform_interface
description: A common platform interface for the file_selector plugin.
repository: https://github.com/flutter/packages/tree/main/packages/file_selector/file_selector_platform_interface
issue_tracker: https://github.com/flutter/flutter/issues?q=is%3Aissue+is%3Aopen+label%3A%22p%3A+file_selector%22
# NOTE: We strongly prefer non-breaking changes, even at the expense of a
# less-clean API. See https://flutter.dev/go/platform-interface-breaking-changes
version: 2.6.2
environment:
sdk: ^3.1.0
flutter: ">=3.13.0"
dependencies:
cross_file: ^0.3.0
flutter:
sdk: flutter
http: ">=0.13.0 <2.0.0"
plugin_platform_interface: ^2.1.7
dev_dependencies:
flutter_test:
sdk: flutter
test: ^1.16.3
topics:
- files
- file-selection
- file-selector
| packages/packages/file_selector/file_selector_platform_interface/pubspec.yaml/0 | {
"file_path": "packages/packages/file_selector/file_selector_platform_interface/pubspec.yaml",
"repo_id": "packages",
"token_count": 310
} | 957 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:js_interop';
import 'package:file_selector_platform_interface/file_selector_platform_interface.dart';
import 'package:flutter/foundation.dart' show visibleForTesting;
import 'package:flutter/services.dart';
import 'package:web/web.dart';
/// Class to manipulate the DOM with the intention of reading files from it.
class DomHelper {
/// Default constructor, initializes the container DOM element.
DomHelper() {
final Element body = document.querySelector('body')!;
body.appendChild(_container);
}
final Element _container = document.createElement('file-selector');
/// Sets the <input /> attributes and waits for a file to be selected.
Future<List<XFile>> getFiles({
String accept = '',
bool multiple = false,
@visibleForTesting HTMLInputElement? input,
}) {
final Completer<List<XFile>> completer = Completer<List<XFile>>();
final HTMLInputElement inputElement =
input ?? (document.createElement('input') as HTMLInputElement)
..type = 'file';
_container.appendChild(
inputElement
..accept = accept
..multiple = multiple,
);
inputElement.onChange.first.then((_) {
final List<XFile> files = Iterable<File>.generate(
inputElement.files!.length,
(int i) => inputElement.files!.item(i)!)
.map(_convertFileToXFile)
.toList();
inputElement.remove();
completer.complete(files);
});
inputElement.onError.first.then((Event event) {
final ErrorEvent error = event as ErrorEvent;
final PlatformException platformException = PlatformException(
code: error.type,
message: error.message,
);
inputElement.remove();
completer.completeError(platformException);
});
inputElement.addEventListener(
'cancel',
(Event event) {
inputElement.remove();
completer.complete(<XFile>[]);
}.toJS,
);
// TODO(dit): Reimplement this with the showPicker() API, https://github.com/flutter/flutter/issues/130365
inputElement.click();
return completer.future;
}
XFile _convertFileToXFile(File file) => XFile(
URL.createObjectURL(file),
name: file.name,
length: file.size,
lastModified: DateTime.fromMillisecondsSinceEpoch(file.lastModified),
);
}
| packages/packages/file_selector/file_selector_web/lib/src/dom_helper.dart/0 | {
"file_path": "packages/packages/file_selector/file_selector_web/lib/src/dom_helper.dart",
"repo_id": "packages",
"token_count": 921
} | 958 |
name: flutter_adaptive_scaffold_example
description: Multiple examples of the usage of the AdaptiveScaffold widget and its lower level widgets.
publish_to: 'none'
version: 0.0.1
environment:
sdk: ^3.1.0
flutter: ">=3.13.0"
dependencies:
flutter:
sdk: flutter
flutter_adaptive_scaffold:
path: ..
dev_dependencies:
build_runner: ^2.1.10
flutter_test:
sdk: flutter
flutter:
uses-material-design: true
assets:
- images/potato.png
- images/habanero.png
- images/strawberry.png
- images/plum.png
- images/mushroom.png
- images/avocado.png
| packages/packages/flutter_adaptive_scaffold/example/pubspec.yaml/0 | {
"file_path": "packages/packages/flutter_adaptive_scaffold/example/pubspec.yaml",
"repo_id": "packages",
"token_count": 242
} | 959 |
name: flutter_adaptive_scaffold
description: Widgets to easily build adaptive layouts, including navigation elements.
version: 0.1.8
issue_tracker: https://github.com/flutter/flutter/issues?q=is%3Aissue+is%3Aopen+label%3A%22p%3A+flutter_adaptive_scaffold%22
repository: https://github.com/flutter/packages/tree/main/packages/flutter_adaptive_scaffold
environment:
sdk: ^3.1.0
flutter: ">=3.13.0"
dependencies:
flutter:
sdk: flutter
dev_dependencies:
flutter_test:
sdk: flutter
topics:
- layout
- ui
| packages/packages/flutter_adaptive_scaffold/pubspec.yaml/0 | {
"file_path": "packages/packages/flutter_adaptive_scaffold/pubspec.yaml",
"repo_id": "packages",
"token_count": 207
} | 960 |
[](https://pub.dev/packages/flutter_lints)
This package contains a recommended set of lints for [Flutter] apps, packages,
and plugins to encourage good coding practices.
This package is built on top of Dart's `recommended.yaml` set of lints from
[package:lints].
Lints are surfaced by the [dart analyzer], which statically checks dart code.
[Dart-enabled IDEs] typically present the issues identified by the analyzer in
their UI. Alternatively, the analyzer can be invoked manually by running
`flutter analyze`.
## Usage
Flutter apps, packages, and plugins created with `flutter create` starting with
Flutter version 2.3.0 are already set up to use the lints defined in this
package. Entities created before that version can use these lints by following
these instructions:
1. Depend on this package as a **dev_dependency** by running
`flutter pub add dev:flutter_lints`.
2. Create an `analysis_options.yaml` file at the root of the package (alongside
the `pubspec.yaml` file) and `include: package:flutter_lints/flutter.yaml`
from it.
Example `analysis_options.yaml` file:
```yaml
# This file configures the analyzer, which statically analyzes Dart code to
# check for errors, warnings, and lints.
#
# The issues identified by the analyzer are surfaced in the UI of Dart-enabled
# IDEs (https://dart.dev/tools#ides-and-editors). The analyzer can also be
# invoked from the command line by running `flutter analyze`.
# The following line activates a set of recommended lints for Flutter apps,
# packages, and plugins designed to encourage good coding practices.
include: package:flutter_lints/flutter.yaml
linter:
# The lint rules applied to this project can be customized in the
# section below to disable rules from the `package:flutter_lints/flutter.yaml`
# included above or to enable additional rules. A list of all available lints
# and their documentation is published at https://dart.dev/lints.
#
# Instead of disabling a lint rule for the entire project in the
# section below, it can also be suppressed for a single line of code
# or a specific dart file by using the `// ignore: name_of_lint` and
# `// ignore_for_file: name_of_lint` syntax on the line or in the file
# producing the lint.
rules:
# avoid_print: false # Uncomment to disable the `avoid_print` rule
# prefer_single_quotes: true # Uncomment to enable the `prefer_single_quotes` rule
# Additional information about this file can be found at
# https://dart.dev/guides/language/analysis-options
```
## Adding new lints
Please file a [lint proposal] issue to suggest that an existing lint rule should
be added to this package. The benefits and risks of adding a lint should be
discussed on that issue with all stakeholders involved. The suggestions will be
reviewed periodically (typically once a year). Following a review, the package
will be updated with all lints that made the cut.
Adding a lint to the package may create new warnings for existing users and is
therefore considered to be a breaking change, which will require a major version
bump. To keep churn low, lints are not added one-by-one, but in one batch
following a review of all accumulated suggestions since the previous review.
[Flutter]: https://flutter.dev
[dart analyzer]: https://dart.dev/guides/language/analysis-options
[Dart-enabled IDEs]: https://dart.dev/tools#ides-and-editors
[package:lints]: https://pub.dev/packages/lints
[lint proposal]: https://github.com/dart-lang/lints/issues/new?&labels=type-lint&template=lint-propoposal.md
| packages/packages/flutter_lints/README.md/0 | {
"file_path": "packages/packages/flutter_lints/README.md",
"repo_id": "packages",
"token_count": 1019
} | 961 |
# flutter_markdown_example
Demonstrates how to use the flutter_markdown package.
| packages/packages/flutter_markdown/example/README.md/0 | {
"file_path": "packages/packages/flutter_markdown/example/README.md",
"repo_id": "packages",
"token_count": 25
} | 962 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// TODO(goderbauer): Restructure the examples to avoid this ignore, https://github.com/flutter/flutter/issues/110208.
// ignore_for_file: avoid_implementing_value_types
import 'package:flutter/material.dart';
import 'package:flutter_markdown/flutter_markdown.dart';
import '../shared/dropdown_menu.dart' as dropdown;
import '../shared/markdown_demo_widget.dart';
// ignore_for_file: public_member_api_docs
const String _data = '''
**MarkdownBody**
''';
const String _notes = '''
# Shrink wrap demo
---
## Overview
This example demonstrates how `MarkdownBody`'s `shrinkWrap` property works.
- If `shrinkWrap` is `true`, `MarkdownBody` will take the minimum height that
wraps its content.
- If `shrinkWrap` is `false`, `MarkdownBody` will expand to the maximum allowed
height.
''';
class MarkdownBodyShrinkWrapDemo extends StatefulWidget
implements MarkdownDemoWidget {
const MarkdownBodyShrinkWrapDemo({super.key});
static const String _title = 'Shrink wrap demo';
@override
String get title => MarkdownBodyShrinkWrapDemo._title;
@override
String get description => "This example demonstrates how MarkdownBody's "
'shrinkWrap property works.';
@override
Future<String> get data => Future<String>.value(_data);
@override
Future<String> get notes => Future<String>.value(_notes);
@override
State<MarkdownBodyShrinkWrapDemo> createState() =>
_MarkdownBodyShrinkWrapDemoState();
}
class _MarkdownBodyShrinkWrapDemoState
extends State<MarkdownBodyShrinkWrapDemo> {
bool _shrinkWrap = true;
final Map<String, bool> _shrinkWrapMenuItems = <String, bool>{
'true': true,
'false': false,
};
@override
Widget build(BuildContext context) {
return Column(
children: <Widget>[
dropdown.DropdownMenu<bool>(
items: _shrinkWrapMenuItems,
label: 'Shrink wrap:',
initialValue: _shrinkWrap,
onChanged: (bool? value) {
if (value != _shrinkWrap) {
setState(() {
_shrinkWrap = value!;
});
}
},
),
Expanded(
child: Stack(
children: <Widget>[
Align(
alignment: Alignment.bottomCenter,
child: Container(
decoration: BoxDecoration(
color: Colors.grey.withOpacity(0.5),
border: Border.all(
color: Colors.red,
width: 2,
),
),
child: MarkdownBody(
data: _data,
shrinkWrap: _shrinkWrap,
),
),
),
],
),
),
],
);
}
}
| packages/packages/flutter_markdown/example/lib/demos/markdown_body_shrink_wrap_demo.dart/0 | {
"file_path": "packages/packages/flutter_markdown/example/lib/demos/markdown_body_shrink_wrap_demo.dart",
"repo_id": "packages",
"token_count": 1307
} | 963 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_markdown/flutter_markdown.dart';
import 'package:flutter_test/flutter_test.dart';
import 'utils.dart';
void main() => defineTests();
void defineTests() {
group('Scrollable', () {
testWidgets(
'code block',
(WidgetTester tester) async {
const String data =
"```\nvoid main() {\n print('Hello World!');\n}\n```";
await tester.pumpWidget(
boilerplate(
const MediaQuery(
data: MediaQueryData(),
child: MarkdownBody(data: data),
),
),
);
final Iterable<Widget> widgets = tester.allWidgets;
final Iterable<SingleChildScrollView> scrollViews =
widgets.whereType<SingleChildScrollView>();
expect(scrollViews, isNotEmpty);
expect(scrollViews.first.controller, isNotNull);
},
);
testWidgets(
'controller',
(WidgetTester tester) async {
final ScrollController controller = ScrollController(
initialScrollOffset: 209.0,
);
await tester.pumpWidget(
boilerplate(
Markdown(controller: controller, data: ''),
),
);
double realOffset() {
return tester
.state<ScrollableState>(find.byType(Scrollable))
.position
.pixels;
}
expect(controller.offset, equals(209.0));
expect(realOffset(), equals(controller.offset));
},
);
testWidgets(
'Scrollable wrapping',
(WidgetTester tester) async {
await tester.pumpWidget(
boilerplate(
const Markdown(data: ''),
),
);
final List<Widget> widgets = selfAndDescendantWidgetsOf(
find.byType(Markdown),
tester,
).toList();
expectWidgetTypes(widgets.take(2), <Type>[
Markdown,
ListView,
]);
expectWidgetTypes(widgets.reversed.take(2).toList().reversed, <Type>[
SliverPadding,
SliverList,
]);
},
);
});
}
| packages/packages/flutter_markdown/test/scrollable_test.dart/0 | {
"file_path": "packages/packages/flutter_markdown/test/scrollable_test.dart",
"repo_id": "packages",
"token_count": 1068
} | 964 |
test_on: vm
| packages/packages/flutter_migrate/dart_test.yaml/0 | {
"file_path": "packages/packages/flutter_migrate/dart_test.yaml",
"repo_id": "packages",
"token_count": 6
} | 965 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:path/path.dart';
import 'base/common.dart';
import 'base/file_system.dart';
import 'base/logger.dart';
import 'base/project.dart';
import 'custom_merge.dart';
import 'environment.dart';
import 'flutter_project_metadata.dart';
import 'migrate_logger.dart';
import 'result.dart';
import 'utils.dart';
// This defines paths of files and directories relative to the project root
// that should be ignored by the migrate tool regardless of .gitignore and
// config settings.
// Paths use `/` as a stand-in for path separator.
const List<String> _skippedFiles = <String>[
'ios/Runner.xcodeproj/project.pbxproj', // Xcode managed configs that may not merge cleanly.
'README.md', // changes to this shouldn't be overwritten since is is user owned.
];
const List<String> _skippedDirectories = <String>[
'.dart_tool', // The .dart_tool generated dir.
'.git', // Git metadata.
'assets', // Common directory for user assets.
'build', // Build artifacts.
'lib', // Always user owned and we don't want to overwrite their apps.
'test', // Typically user owned and flutter-side changes are not relevant.
];
final Iterable<String> canonicalizedSkippedFiles = _skippedFiles.map<String>(
(String path) => canonicalize(path),
);
// Returns true for paths relative to the project root that should be skipped
// completely by the migrate tool.
bool _skipped(String localPath, FileSystem fileSystem,
{Set<String>? skippedPrefixes}) {
final String canonicalizedLocalPath = canonicalize(localPath);
final Iterable<String> canonicalizedSkippedFiles =
_skippedFiles.map<String>((String path) => canonicalize(path));
if (canonicalizedSkippedFiles.contains(canonicalizedLocalPath)) {
return true;
}
final Iterable<String> canonicalizedSkippedDirectories =
_skippedDirectories.map<String>((String path) => canonicalize(path));
for (final String dir in canonicalizedSkippedDirectories) {
if (canonicalizedLocalPath.startsWith('$dir${fileSystem.path.separator}')) {
return true;
}
}
if (skippedPrefixes != null) {
return skippedPrefixes.any((String prefix) => localPath.startsWith(
'${normalize(prefix.replaceAll(r'\', fileSystem.path.separator))}${fileSystem.path.separator}'));
}
return false;
}
// File extensions that the tool will not attempt to merge. Changes
// in files with these extensions will be accepted wholesale.
//
// The executables and binaries in this list are not meant to be
// comprehensive and need only cover the files that are generated
// in `flutter create` as only files generated by the template
// will be attempted to be merged.
const List<String> _doNotMergeFileExtensions = <String>[
// Don't merge image files
'.bmp',
'.gif',
'.jpg',
'.jpeg',
'.png',
'.svg',
// Don't merge compiled artifacts and executables
'.dll',
'.exe',
'.jar',
'.so',
];
// These files should always go through the migrate process as
// they are either integral to the migrate process or we expect
// new versions of this file to always be desired.
const Set<String> _alwaysMigrateFiles = <String>{
'.metadata', // .metadata tracks key migration information.
'android/gradle/wrapper/gradle-wrapper.jar',
// Always add .gitignore back in even if user-deleted as it makes it
// difficult to migrate in the future and the migrate tool enforces git
// usage.
'.gitignore',
};
/// False for files that should not be merged. Typically, images and binary files.
bool _mergable(String localPath) {
return _alwaysMigrateFiles.contains(localPath) ||
!_doNotMergeFileExtensions.any((String ext) => localPath.endsWith(ext));
}
// Compile the set of path prefixes that should be ignored as configured
// in the command arguments.
Set<String> _getSkippedPrefixes(List<SupportedPlatform> platforms) {
final Set<String> skippedPrefixes = <String>{};
for (final SupportedPlatform platform in SupportedPlatform.values) {
skippedPrefixes.add(platformToSubdirectoryPrefix(platform));
}
for (final SupportedPlatform platform in platforms) {
skippedPrefixes.remove(platformToSubdirectoryPrefix(platform));
}
return skippedPrefixes;
}
/// Data class holds the common context that is used throughout the steps of a migrate computation.
class MigrateContext {
MigrateContext({
required this.flutterProject,
required this.skippedPrefixes,
required this.fileSystem,
required this.migrateLogger,
required this.migrateUtils,
required this.environment,
this.baseProject,
this.targetProject,
});
final FlutterProject flutterProject;
final Set<String> skippedPrefixes;
final FileSystem fileSystem;
final MigrateLogger migrateLogger;
final MigrateUtils migrateUtils;
final FlutterToolsEnvironment environment;
MigrateBaseFlutterProject? baseProject;
MigrateTargetFlutterProject? targetProject;
}
/// Returns the path relative to the flutter project's root.
String getLocalPath(String path, String basePath, FileSystem fileSystem) {
return path.replaceFirst(basePath + fileSystem.path.separator, '');
}
String platformToSubdirectoryPrefix(SupportedPlatform platform) {
switch (platform) {
case SupportedPlatform.android:
return 'android';
case SupportedPlatform.ios:
return 'ios';
case SupportedPlatform.linux:
return 'linux';
case SupportedPlatform.macos:
return 'macos';
case SupportedPlatform.web:
return 'web';
case SupportedPlatform.windows:
return 'windows';
case SupportedPlatform.fuchsia:
return 'fuchsia';
}
}
/// Data class that contains the command line arguments passed by the user.
class MigrateCommandParameters {
MigrateCommandParameters({
this.baseAppPath,
this.targetAppPath,
this.baseRevision,
this.targetRevision,
this.preferTwoWayMerge = false,
this.verbose = false,
this.allowFallbackBaseRevision = false,
this.deleteTempDirectories = true,
this.platforms,
});
final String? baseAppPath;
final String? targetAppPath;
final String? baseRevision;
final String? targetRevision;
final bool preferTwoWayMerge;
final bool verbose;
final bool allowFallbackBaseRevision;
final bool deleteTempDirectories;
final List<SupportedPlatform>? platforms;
}
/// Computes the changes that migrates the current flutter project to the target revision.
///
/// This is the entry point to the core migration computations and drives the migration process.
///
/// This method attempts to find a base revision, which is the revision of the Flutter SDK
/// the app was generated with or the last revision the app was migrated to. The base revision
/// typically comes from the .metadata, but for legacy apps, the config may not exist. In
/// this case, we fallback to using the revision in .metadata, and if that does not exist, we
/// use the target revision as the base revision. In the final fallback case, the migration should
/// still work, but will likely generate slightly less accurate merges.
///
/// Operations the computation performs:
///
/// - Parse .metadata file
/// - Collect revisions to use for each platform
/// - Download each flutter revision and call `flutter create` for each.
/// - Call `flutter create` with target revision (target is typically current flutter version)
/// - Diff base revision generated app with target revision generated app
/// - Compute all newly added files between base and target revisions
/// - Compute merge of all files that are modified by user and flutter
/// - Track temp dirs to be deleted
///
/// Structure: This method builds upon a MigrateResult instance
Future<MigrateResult?> computeMigration({
FlutterProject? flutterProject,
required MigrateCommandParameters commandParameters,
required FileSystem fileSystem,
required Logger logger,
required MigrateUtils migrateUtils,
required FlutterToolsEnvironment environment,
}) async {
flutterProject ??= FlutterProject.current(fileSystem);
final MigrateLogger migrateLogger =
MigrateLogger(logger: logger, verbose: commandParameters.verbose);
migrateLogger.logStep('start');
// Find the path prefixes to ignore. This allows subdirectories of platforms
// not part of the migration to be skipped.
final List<SupportedPlatform> platforms =
commandParameters.platforms ?? flutterProject.getSupportedPlatforms();
final Set<String> skippedPrefixes = _getSkippedPrefixes(platforms);
final MigrateResult result = MigrateResult.empty();
final MigrateContext context = MigrateContext(
flutterProject: flutterProject,
skippedPrefixes: skippedPrefixes,
migrateLogger: migrateLogger,
fileSystem: fileSystem,
migrateUtils: migrateUtils,
environment: environment,
);
migrateLogger.logStep('revisions');
final MigrateRevisions revisionConfig = MigrateRevisions(
context: context,
baseRevision: commandParameters.baseRevision,
allowFallbackBaseRevision: commandParameters.allowFallbackBaseRevision,
platforms: platforms,
environment: environment,
);
// Extract the unamanged files/paths that should be ignored by the migrate tool.
// These paths are absolute paths.
migrateLogger.logStep('unmanaged');
final List<String> unmanagedFiles = <String>[];
final List<String> unmanagedDirectories = <String>[];
final String basePath = flutterProject.directory.path;
for (final String localPath in revisionConfig.config.unmanagedFiles) {
if (localPath.endsWith(fileSystem.path.separator)) {
unmanagedDirectories.add(fileSystem.path.join(basePath, localPath));
} else {
unmanagedFiles.add(fileSystem.path.join(basePath, localPath));
}
}
migrateLogger.logStep('generating_base');
// Generate the base templates
final ReferenceProjects referenceProjects =
await _generateBaseAndTargetReferenceProjects(
context: context,
result: result,
revisionConfig: revisionConfig,
platforms: platforms,
commandParameters: commandParameters,
);
// Generate diffs. These diffs are used to determine if a file is newly added, needs merging,
// or deleted (rare). Only files with diffs between the base and target revisions need to be
// migrated. If files are unchanged between base and target, then there are no changes to merge.
migrateLogger.logStep('diff');
result.diffMap.addAll(await referenceProjects.baseProject
.diff(context, referenceProjects.targetProject));
// Check for any new files that were added in the target reference app that did not
// exist in the base reference app.
migrateLogger.logStep('new_files');
result.addedFiles.addAll(await referenceProjects.baseProject
.computeNewlyAddedFiles(
context, result, referenceProjects.targetProject));
// Merge any base->target changed files with the version in the developer's project.
// Files that the developer left unchanged are fully updated to match the target reference.
// Files that the developer changed and were changed from base->target are merged.
migrateLogger.logStep('merging');
await MigrateFlutterProject.merge(
context,
result,
referenceProjects.baseProject,
referenceProjects.targetProject,
unmanagedFiles,
unmanagedDirectories,
commandParameters.preferTwoWayMerge,
);
// Clean up any temp directories generated by this tool.
migrateLogger.logStep('cleaning');
_registerTempDirectoriesForCleaning(
commandParameters: commandParameters,
result: result,
referenceProjects: referenceProjects);
migrateLogger.stop();
return result;
}
/// Returns a base revision to fallback to in case a true base revision is unknown.
String _getFallbackBaseRevision(
bool allowFallbackBaseRevision, MigrateLogger migrateLogger) {
if (!allowFallbackBaseRevision) {
migrateLogger.stop();
migrateLogger.printError(
'Could not determine base revision this app was created with:');
migrateLogger.printError(
'.metadata file did not exist or did not contain a valid revision.',
indent: 2);
migrateLogger.printError(
'Run this command again with the `--allow-fallback-base-revision` flag to use Flutter v1.0.0 as the base revision or manually pass a revision with `--base-revision=<revision>`',
indent: 2);
throwToolExit('Failed to resolve base revision');
}
// Earliest version of flutter with .metadata: c17099f474675d8066fec6984c242d8b409ae985 (2017)
// Flutter 2.0.0: 60bd88df915880d23877bfc1602e8ddcf4c4dd2a
// Flutter v1.0.0: 5391447fae6209bb21a89e6a5a6583cac1af9b4b
//
// TODO(garyq): Use things like dart sdk version and other hints to better fine-tune this fallback.
//
// We fall back on flutter v1.0.0 if .metadata doesn't exist.
migrateLogger.printIfVerbose(
'Could not determine base revision, falling back on `v1.0.0`, revision 5391447fae6209bb21a89e6a5a6583cac1af9b4b');
return '5391447fae6209bb21a89e6a5a6583cac1af9b4b';
}
/// Simple data class that holds the base and target reference
/// projects.
class ReferenceProjects {
ReferenceProjects({
required this.baseProject,
required this.targetProject,
required this.customBaseProjectDir,
required this.customTargetProjectDir,
});
MigrateBaseFlutterProject baseProject;
MigrateTargetFlutterProject targetProject;
// Whether a user provided base and target projects were provided.
bool customBaseProjectDir;
bool customTargetProjectDir;
}
// Generate reference base and target flutter projects.
//
// This function generates reference vaniilla projects by using `flutter create` with
// the base revision Flutter SDK as well as the target revision SDK.
Future<ReferenceProjects> _generateBaseAndTargetReferenceProjects({
required MigrateContext context,
required MigrateResult result,
required MigrateRevisions revisionConfig,
required List<SupportedPlatform> platforms,
required MigrateCommandParameters commandParameters,
}) async {
// Use user-provided projects if provided, if not, generate them internally.
final bool customBaseProjectDir = commandParameters.baseAppPath != null;
final bool customTargetProjectDir = commandParameters.targetAppPath != null;
Directory baseProjectDir =
context.fileSystem.systemTempDirectory.createTempSync('baseProject');
Directory targetProjectDir =
context.fileSystem.systemTempDirectory.createTempSync('targetProject');
if (customBaseProjectDir) {
baseProjectDir =
context.fileSystem.directory(commandParameters.baseAppPath);
} else {
baseProjectDir =
context.fileSystem.systemTempDirectory.createTempSync('baseProject');
context.migrateLogger
.printIfVerbose('Created temporary directory: ${baseProjectDir.path}');
}
if (customTargetProjectDir) {
targetProjectDir =
context.fileSystem.directory(commandParameters.targetAppPath);
} else {
targetProjectDir =
context.fileSystem.systemTempDirectory.createTempSync('targetProject');
context.migrateLogger.printIfVerbose(
'Created temporary directory: ${targetProjectDir.path}');
}
// Git init to enable running further git commands on the reference projects.
await context.migrateUtils.gitInit(baseProjectDir.absolute.path);
await context.migrateUtils.gitInit(targetProjectDir.absolute.path);
result.generatedBaseTemplateDirectory = baseProjectDir;
result.generatedTargetTemplateDirectory = targetProjectDir;
final String name =
context.environment['FlutterProject.manifest.appname']! as String;
final String androidLanguage =
context.environment['FlutterProject.android.isKotlin']! as bool
? 'kotlin'
: 'java';
final String iosLanguage =
context.environment['FlutterProject.ios.isSwift']! as bool
? 'swift'
: 'objc';
final Directory targetFlutterDirectory = context.fileSystem
.directory(context.environment.getString('Cache.flutterRoot'));
// Create the base reference vanilla app.
//
// This step clones the base flutter sdk, and uses it to create a new vanilla app.
// The vanilla base app is used as part of a 3 way merge between the base app, target
// app, and the current user-owned app.
final MigrateBaseFlutterProject baseProject = MigrateBaseFlutterProject(
path: commandParameters.baseAppPath,
directory: baseProjectDir,
name: name,
androidLanguage: androidLanguage,
iosLanguage: iosLanguage,
platformWhitelist: platforms,
);
context.baseProject = baseProject;
await baseProject.createProject(
context,
result,
revisionConfig.revisionsList,
revisionConfig.revisionToConfigs,
commandParameters.baseRevision ??
revisionConfig.metadataRevision ??
_getFallbackBaseRevision(
commandParameters.allowFallbackBaseRevision, context.migrateLogger),
revisionConfig.targetRevision,
targetFlutterDirectory,
);
// Create target reference app when not provided.
//
// This step directly calls flutter create with the target (the current installed revision)
// flutter sdk.
final MigrateTargetFlutterProject targetProject = MigrateTargetFlutterProject(
path: commandParameters.targetAppPath,
directory: targetProjectDir,
name: name,
androidLanguage: androidLanguage,
iosLanguage: iosLanguage,
platformWhitelist: platforms,
);
context.targetProject = targetProject;
await targetProject.createProject(
context,
result,
revisionConfig.targetRevision,
targetFlutterDirectory,
);
return ReferenceProjects(
baseProject: baseProject,
targetProject: targetProject,
customBaseProjectDir: customBaseProjectDir,
customTargetProjectDir: customTargetProjectDir,
);
}
// Registers any generated temporary directories for optional deletion upon tool exit.
void _registerTempDirectoriesForCleaning({
required MigrateCommandParameters commandParameters,
required MigrateResult result,
required ReferenceProjects referenceProjects,
}) {
if (commandParameters.deleteTempDirectories) {
// Don't delete user-provided directories
if (!referenceProjects.customBaseProjectDir) {
result.tempDirectories.add(result.generatedBaseTemplateDirectory!);
}
if (!referenceProjects.customTargetProjectDir) {
result.tempDirectories.add(result.generatedTargetTemplateDirectory!);
}
result.tempDirectories.addAll(result.sdkDirs.values);
}
}
/// A reference flutter project.
///
/// A MigrateFlutterProject is a project that is generated internally within the tool
/// to see what changes need to be made to the user's project. This class
/// provides methods to merge, diff, and otherwise compare multiple MigrateFlutterProject
/// instances.
abstract class MigrateFlutterProject {
MigrateFlutterProject({
required this.path,
required this.directory,
required this.name,
required this.androidLanguage,
required this.iosLanguage,
this.platformWhitelist,
});
final String? path;
final Directory directory;
final String name;
final String androidLanguage;
final String iosLanguage;
final List<SupportedPlatform>? platformWhitelist;
/// Run git diff over each matching pair of files in the this project and the provided target project.
Future<Map<String, DiffResult>> diff(
MigrateContext context,
MigrateFlutterProject other,
) async {
final Map<String, DiffResult> diffMap = <String, DiffResult>{};
final List<FileSystemEntity> thisFiles =
directory.listSync(recursive: true);
int modifiedFilesCount = 0;
for (final FileSystemEntity entity in thisFiles) {
if (entity is! File) {
continue;
}
final File thisFile = entity.absolute;
final String localPath = getLocalPath(
thisFile.path, directory.absolute.path, context.fileSystem);
if (_skipped(localPath, context.fileSystem,
skippedPrefixes: context.skippedPrefixes)) {
continue;
}
if (await context.migrateUtils
.isGitIgnored(thisFile.absolute.path, directory.absolute.path)) {
diffMap[localPath] = DiffResult(diffType: DiffType.ignored);
}
final File otherFile = other.directory.childFile(localPath);
if (otherFile.existsSync()) {
final DiffResult diff =
await context.migrateUtils.diffFiles(thisFile, otherFile);
diffMap[localPath] = diff;
if (diff.diff != '') {
context.migrateLogger.printIfVerbose(
'Found ${diff.exitCode} changes in $localPath',
indent: 4);
modifiedFilesCount++;
}
} else {
// Current file has no new template counterpart, which is equivalent to a deletion.
// This could also indicate a renaming if there is an addition with equivalent contents.
diffMap[localPath] = DiffResult(diffType: DiffType.deletion);
}
}
context.migrateLogger.printIfVerbose(
'$modifiedFilesCount files were modified between base and target apps.');
return diffMap;
}
/// Find all files that exist in the target reference app but not in the base reference app.
Future<List<FilePendingMigration>> computeNewlyAddedFiles(
MigrateContext context,
MigrateResult result,
MigrateFlutterProject other,
) async {
final List<FilePendingMigration> addedFiles = <FilePendingMigration>[];
final List<FileSystemEntity> otherFiles =
other.directory.listSync(recursive: true);
for (final FileSystemEntity entity in otherFiles) {
if (entity is! File) {
continue;
}
final File otherFile = entity.absolute;
final String localPath = getLocalPath(
otherFile.path, other.directory.absolute.path, context.fileSystem);
if (directory.childFile(localPath).existsSync() ||
_skipped(localPath, context.fileSystem,
skippedPrefixes: context.skippedPrefixes)) {
continue;
}
if (await context.migrateUtils.isGitIgnored(
otherFile.absolute.path, other.directory.absolute.path)) {
result.diffMap[localPath] = DiffResult(diffType: DiffType.ignored);
}
result.diffMap[localPath] = DiffResult(diffType: DiffType.addition);
if (context.flutterProject.directory.childFile(localPath).existsSync()) {
// Don't store as added file if file already exists in the project.
continue;
}
addedFiles.add(FilePendingMigration(localPath, otherFile));
}
context.migrateLogger.printIfVerbose(
'${addedFiles.length} files were newly added in the target app.');
return addedFiles;
}
/// Loops through each existing file and intelligently merges it with the base->target changes.
static Future<void> merge(
MigrateContext context,
MigrateResult result,
MigrateFlutterProject baseProject,
MigrateFlutterProject targetProject,
List<String> unmanagedFiles,
List<String> unmanagedDirectories,
bool preferTwoWayMerge,
) async {
final List<CustomMerge> customMerges = <CustomMerge>[
MetadataCustomMerge(logger: context.migrateLogger.logger),
];
// For each existing file in the project, we attempt to 3 way merge if it is changed by the user.
final List<FileSystemEntity> currentFiles =
context.flutterProject.directory.listSync(recursive: true);
final String projectRootPath =
context.flutterProject.directory.absolute.path;
final Set<String> missingAlwaysMigrateFiles =
Set<String>.of(_alwaysMigrateFiles);
for (final FileSystemEntity entity in currentFiles) {
if (entity is! File) {
continue;
}
// check if the file is unmanaged/ignored by the migration tool.
bool ignored = false;
ignored = unmanagedFiles.contains(entity.absolute.path);
for (final String path in unmanagedDirectories) {
if (entity.absolute.path.startsWith(path)) {
ignored = true;
break;
}
}
if (ignored) {
continue; // Skip if marked as unmanaged
}
final File currentFile = entity.absolute;
// Diff the current file against the old generated template
final String localPath =
getLocalPath(currentFile.path, projectRootPath, context.fileSystem);
missingAlwaysMigrateFiles.remove(localPath);
if (result.diffMap.containsKey(localPath) &&
result.diffMap[localPath]!.diffType == DiffType.ignored ||
await context.migrateUtils.isGitIgnored(currentFile.path,
context.flutterProject.directory.absolute.path) ||
_skipped(localPath, context.fileSystem,
skippedPrefixes: context.skippedPrefixes) ||
!_mergable(localPath)) {
continue;
}
final File baseTemplateFile = baseProject.directory.childFile(localPath);
final File targetTemplateFile =
targetProject.directory.childFile(localPath);
final DiffResult userDiff =
await context.migrateUtils.diffFiles(currentFile, baseTemplateFile);
final DiffResult targetDiff =
await context.migrateUtils.diffFiles(currentFile, targetTemplateFile);
if (targetDiff.exitCode == 0) {
// current file is already the same as the target file.
continue;
}
final bool alwaysMigrate = _alwaysMigrateFiles.contains(localPath);
// Current file unchanged by user, thus we consider it owned by the tool.
if (userDiff.exitCode == 0 || alwaysMigrate) {
if ((result.diffMap.containsKey(localPath) || alwaysMigrate) &&
result.diffMap[localPath] != null) {
// File changed between base and target
if (result.diffMap[localPath]!.diffType == DiffType.deletion) {
// File is deleted in new template
result.deletedFiles
.add(FilePendingMigration(localPath, currentFile));
continue;
}
if (result.diffMap[localPath]!.exitCode != 0 || alwaysMigrate) {
// Accept the target version wholesale
MergeResult mergeResult;
try {
mergeResult = StringMergeResult.explicit(
mergedString: targetTemplateFile.readAsStringSync(),
hasConflict: false,
exitCode: 0,
localPath: localPath,
);
} on FileSystemException {
mergeResult = BinaryMergeResult.explicit(
mergedBytes: targetTemplateFile.readAsBytesSync(),
hasConflict: false,
exitCode: 0,
localPath: localPath,
);
}
result.mergeResults.add(mergeResult);
continue;
}
}
continue;
}
// File changed by user
if (result.diffMap.containsKey(localPath)) {
MergeResult? mergeResult;
// Default to two way merge as it does not require the base file to exist.
MergeType mergeType =
result.mergeTypeMap[localPath] ?? MergeType.twoWay;
for (final CustomMerge customMerge in customMerges) {
if (customMerge.localPath == localPath) {
mergeResult = customMerge.merge(
currentFile, baseTemplateFile, targetTemplateFile);
mergeType = MergeType.custom;
break;
}
}
if (mergeResult == null) {
late String basePath;
late String currentPath;
late String targetPath;
// Use two way merge if diff between base and target are the same.
// This prevents the three way merge re-deleting the base->target changes.
if (preferTwoWayMerge) {
mergeType = MergeType.twoWay;
}
switch (mergeType) {
case MergeType.twoWay:
{
basePath = currentFile.path;
currentPath = currentFile.path;
targetPath = context.fileSystem.path.join(
result.generatedTargetTemplateDirectory!.path, localPath);
break;
}
case MergeType.threeWay:
{
basePath = context.fileSystem.path.join(
result.generatedBaseTemplateDirectory!.path, localPath);
currentPath = currentFile.path;
targetPath = context.fileSystem.path.join(
result.generatedTargetTemplateDirectory!.path, localPath);
break;
}
case MergeType.custom:
{
break; // handled above
}
}
if (mergeType != MergeType.custom) {
mergeResult = await context.migrateUtils.gitMergeFile(
base: basePath,
current: currentPath,
target: targetPath,
localPath: localPath,
);
}
}
if (mergeResult != null) {
// Don't include if result is identical to the current file.
if (mergeResult is StringMergeResult) {
if (mergeResult.mergedString == currentFile.readAsStringSync()) {
context.migrateLogger
.printIfVerbose('$localPath was merged with a $mergeType.');
continue;
}
} else {
if ((mergeResult as BinaryMergeResult).mergedBytes ==
currentFile.readAsBytesSync()) {
continue;
}
}
result.mergeResults.add(mergeResult);
}
context.migrateLogger
.printStatus('$localPath was merged with a $mergeType.');
continue;
}
}
// Add files that are in the target, marked as always migrate, and missing in the current project.
for (final String localPath in missingAlwaysMigrateFiles) {
final File targetTemplateFile =
result.generatedTargetTemplateDirectory!.childFile(localPath);
if (targetTemplateFile.existsSync() &&
!_skipped(localPath, context.fileSystem,
skippedPrefixes: context.skippedPrefixes)) {
result.addedFiles
.add(FilePendingMigration(localPath, targetTemplateFile));
}
}
}
}
/// The base reference project used in a migration computation.
///
/// This project is a clean re-generation of the version the user's project
/// was 1. originally generated with, or 2. the last successful migrated to.
class MigrateBaseFlutterProject extends MigrateFlutterProject {
MigrateBaseFlutterProject({
required super.path,
required super.directory,
required super.name,
required super.androidLanguage,
required super.iosLanguage,
super.platformWhitelist,
});
/// Creates the base reference app based off of the migrate config in the .metadata file.
Future<void> createProject(
MigrateContext context,
MigrateResult result,
List<String> revisionsList,
Map<String, List<MigratePlatformConfig>> revisionToConfigs,
String fallbackRevision,
String targetRevision,
Directory targetFlutterDirectory,
) async {
// Create base
// Clone base flutter
if (path == null) {
final Map<String, Directory> revisionToFlutterSdkDir =
<String, Directory>{};
for (final String revision in revisionsList) {
final List<String> platforms = <String>[];
for (final MigratePlatformConfig config
in revisionToConfigs[revision]!) {
if (config.component == FlutterProjectComponent.root) {
continue;
}
platforms.add(config.component.toString().split('.').last);
}
// In the case of the revision being invalid or not a hash of the master branch,
// we want to fallback in the following order:
// - parsed revision
// - fallback revision
// - target revision (currently installed flutter)
late Directory sdkDir;
final List<String> revisionsToTry = <String>[revision];
if (revision != fallbackRevision) {
revisionsToTry.add(fallbackRevision);
}
bool sdkAvailable = false;
int index = 0;
do {
if (index < revisionsToTry.length) {
final String activeRevision = revisionsToTry[index++];
if (activeRevision != revision &&
revisionToFlutterSdkDir.containsKey(activeRevision)) {
sdkDir = revisionToFlutterSdkDir[activeRevision]!;
revisionToFlutterSdkDir[revision] = sdkDir;
sdkAvailable = true;
} else {
sdkDir = context.fileSystem.systemTempDirectory
.createTempSync('flutter_$activeRevision');
result.sdkDirs[activeRevision] = sdkDir;
context.migrateLogger.printStatus('Cloning SDK $activeRevision');
sdkAvailable = await context.migrateUtils
.cloneFlutter(activeRevision, sdkDir.absolute.path);
revisionToFlutterSdkDir[revision] = sdkDir;
}
} else {
// fallback to just using the modern target version of flutter.
sdkDir = targetFlutterDirectory;
revisionToFlutterSdkDir[revision] = sdkDir;
sdkAvailable = true;
}
} while (!sdkAvailable);
context.migrateLogger.printStatus(
'Creating base app for $platforms with revision $revision.');
final String newDirectoryPath =
await context.migrateUtils.createFromTemplates(
sdkDir.childDirectory('bin').absolute.path,
name: name,
androidLanguage: androidLanguage,
iosLanguage: iosLanguage,
outputDirectory: result.generatedBaseTemplateDirectory!.absolute.path,
platforms: platforms,
);
if (newDirectoryPath != result.generatedBaseTemplateDirectory?.path) {
result.generatedBaseTemplateDirectory =
context.fileSystem.directory(newDirectoryPath);
}
// Determine merge type for each newly generated file.
final List<FileSystemEntity> generatedBaseFiles =
result.generatedBaseTemplateDirectory!.listSync(recursive: true);
for (final FileSystemEntity entity in generatedBaseFiles) {
if (entity is! File) {
continue;
}
final File baseTemplateFile = entity.absolute;
final String localPath = getLocalPath(
baseTemplateFile.path,
result.generatedBaseTemplateDirectory!.absolute.path,
context.fileSystem);
if (!result.mergeTypeMap.containsKey(localPath)) {
// Use two way merge when the base revision is the same as the target revision.
result.mergeTypeMap[localPath] = revision == targetRevision
? MergeType.twoWay
: MergeType.threeWay;
}
}
if (newDirectoryPath != result.generatedBaseTemplateDirectory?.path) {
result.generatedBaseTemplateDirectory =
context.fileSystem.directory(newDirectoryPath);
break; // The create command is old and does not distinguish between platforms so it only needs to be called once.
}
}
}
}
}
/// Represents a manifested flutter project that is the migration target.
///
/// The files in this project are the version the migrate tool will try
/// to transform the existing files into.
class MigrateTargetFlutterProject extends MigrateFlutterProject {
MigrateTargetFlutterProject({
required super.path,
required super.directory,
required super.name,
required super.androidLanguage,
required super.iosLanguage,
super.platformWhitelist,
});
/// Creates the base reference app based off of the migrate config in the .metadata file.
Future<void> createProject(
MigrateContext context,
MigrateResult result,
String targetRevision,
Directory targetFlutterDirectory,
) async {
if (path == null) {
// Create target
context.migrateLogger
.printStatus('Creating target app with revision $targetRevision.');
context.migrateLogger.printIfVerbose('Creating target app.');
await context.migrateUtils.createFromTemplates(
targetFlutterDirectory.childDirectory('bin').absolute.path,
name: name,
androidLanguage: androidLanguage,
iosLanguage: iosLanguage,
outputDirectory: result.generatedTargetTemplateDirectory!.absolute.path,
);
}
}
}
/// Parses the metadata of the flutter project, extracts, computes, and stores the
/// revisions that the migration should use to migrate between.
class MigrateRevisions {
MigrateRevisions({
required MigrateContext context,
required String? baseRevision,
required bool allowFallbackBaseRevision,
required List<SupportedPlatform> platforms,
required FlutterToolsEnvironment environment,
}) {
_computeRevisions(context, baseRevision, allowFallbackBaseRevision,
platforms, environment);
}
late List<String> revisionsList;
late Map<String, List<MigratePlatformConfig>> revisionToConfigs;
late String fallbackRevision;
late String targetRevision;
late String? metadataRevision;
late MigrateConfig config;
void _computeRevisions(
MigrateContext context,
String? baseRevision,
bool allowFallbackBaseRevision,
List<SupportedPlatform> platforms,
FlutterToolsEnvironment environment,
) {
final List<FlutterProjectComponent> components =
<FlutterProjectComponent>[];
for (final SupportedPlatform platform in platforms) {
components.add(platform.toFlutterProjectComponent());
}
components.add(FlutterProjectComponent.root);
final FlutterProjectMetadata metadata = FlutterProjectMetadata(
context.flutterProject.directory.childFile('.metadata'),
context.migrateLogger.logger);
config = metadata.migrateConfig;
// We call populate in case MigrateConfig is empty. If it is filled, populate should not do anything.
config.populate(
projectDirectory: context.flutterProject.directory,
update: false,
logger: context.migrateLogger.logger,
);
metadataRevision = metadata.versionRevision;
if (environment.getString('FlutterVersion.frameworkRevision') == null) {
throwToolExit('Flutter framework revision was null');
}
targetRevision = environment.getString('FlutterVersion.frameworkRevision')!;
String rootBaseRevision = '';
revisionToConfigs = <String, List<MigratePlatformConfig>>{};
final Set<String> revisions = <String>{};
if (baseRevision == null) {
for (final MigratePlatformConfig platform
in config.platformConfigs.values) {
final String effectiveRevision = platform.baseRevision == null
? metadataRevision ??
_getFallbackBaseRevision(
allowFallbackBaseRevision, context.migrateLogger)
: platform.baseRevision!;
if (!components.contains(platform.component)) {
continue;
}
if (platform.component == FlutterProjectComponent.root) {
rootBaseRevision = effectiveRevision;
}
revisions.add(effectiveRevision);
if (revisionToConfigs[effectiveRevision] == null) {
revisionToConfigs[effectiveRevision] = <MigratePlatformConfig>[];
}
revisionToConfigs[effectiveRevision]!.add(platform);
}
} else {
rootBaseRevision = baseRevision;
revisionToConfigs[baseRevision] = <MigratePlatformConfig>[];
for (final FlutterProjectComponent component in components) {
revisionToConfigs[baseRevision]!.add(MigratePlatformConfig(
component: component, baseRevision: baseRevision));
}
// revisionToConfigs[baseRevision]!.add(
// MigratePlatformConfig(platform: null, baseRevision: baseRevision));
}
// Reorder such that the root revision is created first.
revisions.remove(rootBaseRevision);
revisionsList = List<String>.from(revisions);
if (rootBaseRevision != '') {
revisionsList.insert(0, rootBaseRevision);
}
context.migrateLogger
.printIfVerbose('Potential base revisions: $revisionsList');
fallbackRevision = _getFallbackBaseRevision(true, context.migrateLogger);
if (revisionsList.contains(fallbackRevision) &&
baseRevision != fallbackRevision &&
metadataRevision != fallbackRevision) {
context.migrateLogger.printStatus(
'Using Flutter v1.0.0 ($fallbackRevision) as the base revision since a valid base revision could not be found in the .metadata file. This may result in more merge conflicts than normally expected.',
indent: 4);
}
}
}
| packages/packages/flutter_migrate/lib/src/compute.dart/0 | {
"file_path": "packages/packages/flutter_migrate/lib/src/compute.dart",
"repo_id": "packages",
"token_count": 14270
} | 966 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
@Timeout(Duration(seconds: 600))
library;
import 'dart:io';
import 'package:file/file.dart';
import 'package:flutter_migrate/src/base/file_system.dart';
import '../src/common.dart';
import '../src/test_utils.dart';
import 'project.dart';
class MigrateProject extends Project {
MigrateProject(this.version, {this.vanilla = true, this.main});
final String version;
/// Manually set main.dart
@override
final String? main;
/// Non-vanilla is a set of changed files that guarantee a merge conflict.
final bool vanilla;
late String _appPath;
static Future<void> installProject(String verison, Directory dir,
{bool vanilla = true, String? main}) async {
final MigrateProject project =
MigrateProject(verison, vanilla: vanilla, main: main);
await project.setUpIn(dir);
// Init a git repo to test uncommitted changes checks
await processManager.run(<String>[
'git',
'init',
], workingDirectory: dir.path);
await processManager.run(<String>[
'git',
'checkout',
'-b',
'master',
], workingDirectory: dir.path);
await commitChanges(dir);
}
static Future<void> commitChanges(Directory dir) async {
await processManager.run(<String>[
'git',
'add',
'.',
], workingDirectory: dir.path);
await processManager.run(<String>[
'git',
'commit',
'-m',
'"All changes"',
], workingDirectory: dir.path);
}
@override
Future<void> setUpIn(
Directory dir, {
bool useSyntheticPackage = false,
}) async {
this.dir = dir;
_appPath = dir.path;
writeFile(fileSystem.path.join(dir.path, 'android', 'local.properties'),
androidLocalProperties);
final Directory tempDir = createResolvedTempDirectorySync('cipd_dest.');
final Directory depotToolsDir =
createResolvedTempDirectorySync('depot_tools.');
await processManager.run(<String>[
'git',
'clone',
'https://chromium.googlesource.com/chromium/tools/depot_tools',
depotToolsDir.path,
], workingDirectory: dir.path);
final File cipdFile =
depotToolsDir.childFile(Platform.isWindows ? 'cipd.bat' : 'cipd');
await processManager.run(<String>[
cipdFile.path,
'init',
tempDir.path,
'-force',
], workingDirectory: dir.path);
await processManager.run(<String>[
cipdFile.path,
'install',
'flutter/test/full_app_fixtures/vanilla',
version,
'-root',
tempDir.path,
], workingDirectory: dir.path);
if (Platform.isWindows) {
ProcessResult res = await processManager.run(<String>[
'robocopy',
tempDir.path,
dir.path,
'*',
'/E',
'/V',
'/mov',
]);
// Robocopy exit code 1 means some files were copied. 0 means no files were copied.
assert(res.exitCode == 1);
res = await processManager.run(<String>[
'takeown',
'/f',
dir.path,
'/r',
]);
res = await processManager.run(<String>[
'takeown',
'/f',
'${dir.path}\\lib\\main.dart',
'/r',
]);
res = await processManager.run(<String>[
'icacls',
dir.path,
], workingDirectory: dir.path);
// Add full access permissions to Users
res = await processManager.run(<String>[
'icacls',
dir.path,
'/q',
'/c',
'/t',
'/grant',
'Users:F',
]);
} else {
// This cp command changes the symlinks to real files so the tool can edit them.
await processManager.run(<String>[
'cp',
'-R',
'-L',
'-f',
'${tempDir.path}/.',
dir.path,
]);
await processManager.run(<String>[
'rm',
'-rf',
'.cipd',
], workingDirectory: dir.path);
await processManager.run(<String>[
'chmod',
'-R',
'+w',
dir.path,
], workingDirectory: dir.path);
await processManager.run(<String>[
'chmod',
'-R',
'+r',
dir.path,
], workingDirectory: dir.path);
}
if (!vanilla) {
writeFile(fileSystem.path.join(dir.path, 'lib', 'main.dart'), libMain);
writeFile(fileSystem.path.join(dir.path, 'lib', 'other.dart'), libOther);
writeFile(fileSystem.path.join(dir.path, 'pubspec.yaml'), pubspecCustom);
}
if (main != null) {
writeFile(fileSystem.path.join(dir.path, 'lib', 'main.dart'), main!);
}
tryToDelete(tempDir);
tryToDelete(depotToolsDir);
}
// Maintain the same pubspec as the configured app.
@override
String get pubspec => fileSystem
.file(fileSystem.path.join(_appPath, 'pubspec.yaml'))
.readAsStringSync();
String get androidLocalProperties => '''
flutter.sdk=${getFlutterRoot()}
''';
String get libMain => '''
import 'package:flutter/material.dart';
import 'other.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: OtherWidget(),
);
}
}
''';
String get libOther => '''
class OtherWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Container(width: 100, height: 100);
}
}
''';
String get pubspecCustom => '''
name: vanilla_app_1_22_6_stable
description: This is a modified description from the default.
# The following line prevents the package from being accidentally published to
# pub.dev using `pub publish`. This is preferred for private packages.
publish_to: 'none' # Remove this line if you wish to publish to pub.dev
# The following defines the version and build number for your application.
# A version number is three numbers separated by dots, like 1.2.43
# followed by an optional build number separated by a +.
# Both the version and the builder number may be overridden in flutter
# build by specifying --build-name and --build-number, respectively.
# In Android, build-name is used as versionName while build-number used as versionCode.
# Read more about Android versioning at https://developer.android.com/studio/publish/versioning
# In iOS, build-name is used as CFBundleShortVersionString while build-number used as CFBundleVersion.
# Read more about iOS versioning at
# https://developer.apple.com/library/archive/documentation/General/Reference/InfoPlistKeyReference/Articles/CoreFoundationKeys.html
version: 1.0.0+1
environment:
sdk: ">=2.6.0 <3.0.0"
dependencies:
flutter:
sdk: flutter
# The following adds the Cupertino Icons font to your application.
# Use with the CupertinoIcons class for iOS style icons.
cupertino_icons: ^1.0.0
dev_dependencies:
flutter_test:
sdk: flutter
# For information on the generic Dart part of this file, see the
# following page: https://dart.dev/tools/pub/pubspec
# The following section is specific to Flutter.
flutter:
# The following line ensures that the Material Icons font is
# included with your application, so that you can use the icons in
# the material Icons class.
uses-material-design: true
# To add assets to your application, add an assets section, like this:
assets:
- images/a_dot_burr.jpeg
- images/a_dot_ham.jpeg
# An image asset can refer to one or more resolution-specific "variants", see
# https://flutter.dev/assets-and-images/#resolution-aware.
# For details regarding adding assets from package dependencies, see
# https://flutter.dev/assets-and-images/#from-packages
# To add custom fonts to your application, add a fonts section here,
# in this "flutter" section. Each entry in this list should have a
# "family" key with the font family name, and a "fonts" key with a
# list giving the asset and other descriptors for the font. For
# example:
# fonts:
# - family: Schyler
# fonts:
# - asset: fonts/Schyler-Regular.ttf
# - asset: fonts/Schyler-Italic.ttf
# style: italic
# - family: Trajan Pro
# fonts:
# - asset: fonts/TrajanPro.ttf
# - asset: fonts/TrajanPro_Bold.ttf
# weight: 700
#
# For details regarding fonts from package dependencies,
# see https://flutter.dev/custom-fonts/#from-packages
''';
}
| packages/packages/flutter_migrate/test/test_data/migrate_project.dart/0 | {
"file_path": "packages/packages/flutter_migrate/test/test_data/migrate_project.dart",
"repo_id": "packages",
"token_count": 3303
} | 967 |
# flutter\_template\_images
Images used by the `flutter_tools` templates.
This project is an internal dependency of the `flutter` tool, and is
not intended to be used directly. It contains images files used in
`flutter create` templates, to avoid checking them into [the main
Flutter repository](https://github.com/flutter/flutter), where they would
permanently increase the checkout size over time if altered.
| packages/packages/flutter_template_images/README.md/0 | {
"file_path": "packages/packages/flutter_template_images/README.md",
"repo_id": "packages",
"token_count": 105
} | 968 |
There are many ways to navigate between destinations in your app.
## Go directly to a destination
Navigating to a destination in GoRouter will replace the current stack of screens with the screens configured to be displayed
for the destination route. To change to a new screen, call `context.go()` with a URL:
```
build(BuildContext context) {
return TextButton(
onPressed: () => context.go('/users/123'),
);
}
```
This is shorthand for calling `GoRouter.of(context).go('/users/123)`.
To build a URI with query parameters, you can use the `Uri` class from the Dart standard library:
```
context.go(Uri(path: '/users/123', queryParameters: {'filter': 'abc'}).toString());
```
## Imperative navigation
GoRouter can push a screen onto the Navigator's history
stack using `context.push()`, and can pop the current screen via
`context.pop()`. However, imperative navigation is known to cause issues with
the browser history.
To learn more, see [issue
#99112](https://github.com/flutter/flutter/issues/99112).
## Using the Link widget
You can use a Link widget from the url_launcher package to create a link to destinations in
your app. This is equivalent to calling `context.go()`, but renders a real link
on the web.
To add a Link to your app, follow the [Link API
documentation](https://pub.dev/documentation/url_launcher/latest/link/Link-class.html)
from the url_launcher package.
## Using named routes
You can also use [Named routes] to navigate instead of using URLs.
## Prevent navigation
GoRouter and other Router-based APIs are not compatible with the
[WillPopScope](https://api.flutter.dev/flutter/widgets/WillPopScope-class.html)
widget.
See [issue #102408](https://github.com/flutter/flutter/issues/102408)
for details on what such an API might look like in go_router.
## Imperative navigation with Navigator
You can continue using the Navigator to push and pop pages. Pages displayed in
this way are not deep-linkable and will be replaced if any parent page that is
associated with a GoRoute is removed, for example when a new call to `go()`
occurs.
To push a screen using the imperative Navigator API, call
[`NavigatorState.push()`](https://api.flutter.dev/flutter/widgets/NavigatorState/push.html):
```dart
Navigator.of(context).push(
MaterialPageRoute(
builder: (BuildContext context) {
return const DetailsScreen();
},
),
);
```
The behavior may change depends on the shell route in current screen and the new screen.
If pushing a new screen without any shell route onto the current screen with shell route, the new
screen is placed entirely on top of the current screen.
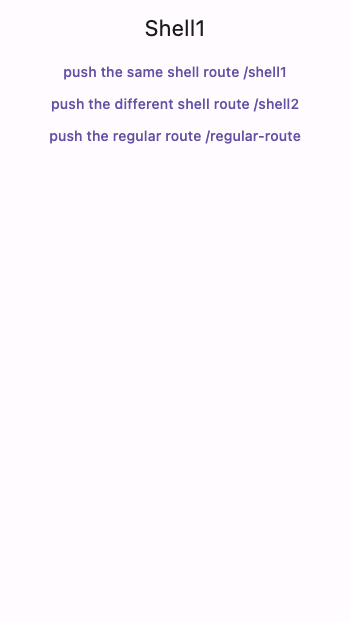
If pushing a new screen with the same shell route as the current screen, the new
screen is placed inside of the shell.
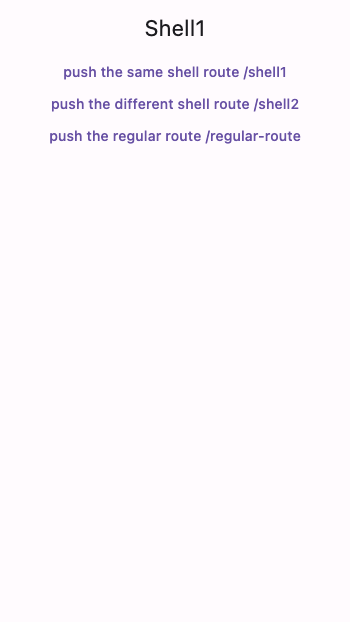
If pushing a new screen with the different shell route as the current screen, the new
screen along with the shell is placed entirely on top of the current screen.
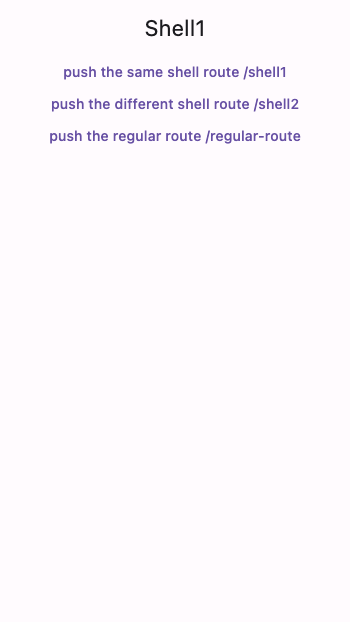
To try out the behavior yourself, see
[push_with_shell_route.dart](https://github.com/flutter/packages/blob/main/packages/go_router/example/lib/extra_codec.dart).
## Returning values
Waiting for a value to be returned:
```dart
onTap: () {
final bool? result = await context.push<bool>('/page2');
if(result ?? false)...
}
```
Returning a value:
```dart
onTap: () => context.pop(true)
```
## Using extra
You can provide additional data along with navigation.
```dart
context.go('/123', extra: 'abc');
```
and retrieve the data from GoRouterState
```dart
final String extraString = GoRouterState.of(context).extra! as String;
```
The extra data will go through serialization when it is stored in the browser.
If you plan to use complex data as extra, consider also providing a codec
to GoRouter so that it won't get dropped during serialization.
For an example on how to use complex data in extra with a codec, see
[extra_codec.dart](https://github.com/flutter/packages/blob/main/packages/go_router/example/lib/extra_codec.dart).
[Named routes]: https://pub.dev/documentation/go_router/latest/topics/Named%20routes-topic.html
| packages/packages/go_router/doc/navigation.md/0 | {
"file_path": "packages/packages/go_router/doc/navigation.md",
"repo_id": "packages",
"token_count": 1325
} | 969 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:go_router/go_router.dart';
import 'package:url_launcher/link.dart';
import '../auth.dart';
/// The settings screen.
class SettingsScreen extends StatefulWidget {
/// Creates a [SettingsScreen].
const SettingsScreen({super.key});
@override
State<SettingsScreen> createState() => _SettingsScreenState();
}
class _SettingsScreenState extends State<SettingsScreen> {
@override
Widget build(BuildContext context) => Scaffold(
body: SafeArea(
child: SingleChildScrollView(
child: Align(
alignment: Alignment.topCenter,
child: ConstrainedBox(
constraints: const BoxConstraints(maxWidth: 400),
child: const Card(
child: Padding(
padding: EdgeInsets.symmetric(vertical: 18, horizontal: 12),
child: SettingsContent(),
),
),
),
),
),
),
);
}
/// The content of a [SettingsScreen].
class SettingsContent extends StatelessWidget {
/// Creates a [SettingsContent].
const SettingsContent({
super.key,
});
@override
Widget build(BuildContext context) => Column(
children: <Widget>[
...<Widget>[
Text(
'Settings',
style: Theme.of(context).textTheme.headlineMedium,
),
ElevatedButton(
onPressed: () {
BookstoreAuthScope.of(context).signOut();
},
child: const Text('Sign out'),
),
Link(
uri: Uri.parse('/book/0'),
builder: (BuildContext context, FollowLink? followLink) =>
TextButton(
onPressed: followLink,
child: const Text('Go directly to /book/0 (Link)'),
),
),
TextButton(
onPressed: () {
context.go('/book/0');
},
child: const Text('Go directly to /book/0 (GoRouter)'),
),
].map<Widget>((Widget w) =>
Padding(padding: const EdgeInsets.all(8), child: w)),
TextButton(
onPressed: () => showDialog<String>(
context: context,
builder: (BuildContext context) => AlertDialog(
title: const Text('Alert!'),
content: const Text('The alert description goes here.'),
actions: <Widget>[
TextButton(
onPressed: () => Navigator.pop(context, 'Cancel'),
child: const Text('Cancel'),
),
TextButton(
onPressed: () => Navigator.pop(context, 'OK'),
child: const Text('OK'),
),
],
),
),
child: const Text('Show Dialog'),
)
],
);
}
| packages/packages/go_router/example/lib/books/src/screens/settings.dart/0 | {
"file_path": "packages/packages/go_router/example/lib/books/src/screens/settings.dart",
"repo_id": "packages",
"token_count": 1589
} | 970 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:go_router/go_router.dart';
void main() => runApp(
const RootRestorationScope(restorationId: 'root', child: App()),
);
/// The main app.
class App extends StatefulWidget {
/// Creates an [App].
const App({super.key});
/// The title of the app.
static const String title = 'GoRouter Example: State Restoration';
@override
State<App> createState() => _AppState();
}
class _AppState extends State<App> with RestorationMixin {
@override
String get restorationId => 'wrapper';
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
// Implement restoreState for your app
}
@override
Widget build(BuildContext context) => MaterialApp.router(
routerConfig: _router,
title: App.title,
restorationScopeId: 'app',
);
final GoRouter _router = GoRouter(
routes: <GoRoute>[
// restorationId set for the route automatically
GoRoute(
path: '/',
builder: (BuildContext context, GoRouterState state) =>
const Page1Screen(),
),
// restorationId set for the route automatically
GoRoute(
path: '/page2',
builder: (BuildContext context, GoRouterState state) =>
const Page2Screen(),
),
],
restorationScopeId: 'router',
);
}
/// The screen of the first page.
class Page1Screen extends StatelessWidget {
/// Creates a [Page1Screen].
const Page1Screen({super.key});
@override
Widget build(BuildContext context) => Scaffold(
appBar: AppBar(title: const Text(App.title)),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
onPressed: () => context.go('/page2'),
child: const Text('Go to page 2'),
),
],
),
),
);
}
/// The screen of the second page.
class Page2Screen extends StatelessWidget {
/// Creates a [Page2Screen].
const Page2Screen({super.key});
@override
Widget build(BuildContext context) => Scaffold(
appBar: AppBar(title: const Text(App.title)),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
onPressed: () => context.go('/'),
child: const Text('Go to home page'),
),
],
),
),
);
}
| packages/packages/go_router/example/lib/others/state_restoration.dart/0 | {
"file_path": "packages/packages/go_router/example/lib/others/state_restoration.dart",
"repo_id": "packages",
"token_count": 1112
} | 971 |
#include "../../Flutter/Flutter-Release.xcconfig"
#include "Warnings.xcconfig"
| packages/packages/go_router/example/macos/Runner/Configs/Release.xcconfig/0 | {
"file_path": "packages/packages/go_router/example/macos/Runner/Configs/Release.xcconfig",
"repo_id": "packages",
"token_count": 32
} | 972 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import '../router.dart';
/// Dart extension to add navigation function to a BuildContext object, e.g.
/// context.go('/');
extension GoRouterHelper on BuildContext {
/// Get a location from route name and parameters.
///
/// This method can't be called during redirects.
String namedLocation(
String name, {
Map<String, String> pathParameters = const <String, String>{},
Map<String, dynamic> queryParameters = const <String, dynamic>{},
}) =>
GoRouter.of(this).namedLocation(name,
pathParameters: pathParameters, queryParameters: queryParameters);
/// Navigate to a location.
void go(String location, {Object? extra}) =>
GoRouter.of(this).go(location, extra: extra);
/// Navigate to a named route.
void goNamed(
String name, {
Map<String, String> pathParameters = const <String, String>{},
Map<String, dynamic> queryParameters = const <String, dynamic>{},
Object? extra,
}) =>
GoRouter.of(this).goNamed(
name,
pathParameters: pathParameters,
queryParameters: queryParameters,
extra: extra,
);
/// Push a location onto the page stack.
///
/// See also:
/// * [pushReplacement] which replaces the top-most page of the page stack and
/// always uses a new page key.
/// * [replace] which replaces the top-most page of the page stack but treats
/// it as the same page. The page key will be reused. This will preserve the
/// state and not run any page animation.
Future<T?> push<T extends Object?>(String location, {Object? extra}) =>
GoRouter.of(this).push<T>(location, extra: extra);
/// Navigate to a named route onto the page stack.
Future<T?> pushNamed<T extends Object?>(
String name, {
Map<String, String> pathParameters = const <String, String>{},
Map<String, dynamic> queryParameters = const <String, dynamic>{},
Object? extra,
}) =>
GoRouter.of(this).pushNamed<T>(
name,
pathParameters: pathParameters,
queryParameters: queryParameters,
extra: extra,
);
/// Returns `true` if there is more than 1 page on the stack.
bool canPop() => GoRouter.of(this).canPop();
/// Pop the top page off the Navigator's page stack by calling
/// [Navigator.pop].
void pop<T extends Object?>([T? result]) => GoRouter.of(this).pop(result);
/// Replaces the top-most page of the page stack with the given URL location
/// w/ optional query parameters, e.g. `/family/f2/person/p1?color=blue`.
///
/// See also:
/// * [go] which navigates to the location.
/// * [push] which pushes the given location onto the page stack.
/// * [replace] which replaces the top-most page of the page stack but treats
/// it as the same page. The page key will be reused. This will preserve the
/// state and not run any page animation.
void pushReplacement(String location, {Object? extra}) =>
GoRouter.of(this).pushReplacement(location, extra: extra);
/// Replaces the top-most page of the page stack with the named route w/
/// optional parameters, e.g. `name='person', pathParameters={'fid': 'f2', 'pid':
/// 'p1'}`.
///
/// See also:
/// * [goNamed] which navigates a named route.
/// * [pushNamed] which pushes a named route onto the page stack.
void pushReplacementNamed(
String name, {
Map<String, String> pathParameters = const <String, String>{},
Map<String, dynamic> queryParameters = const <String, dynamic>{},
Object? extra,
}) =>
GoRouter.of(this).pushReplacementNamed(
name,
pathParameters: pathParameters,
queryParameters: queryParameters,
extra: extra,
);
/// Replaces the top-most page of the page stack with the given one but treats
/// it as the same page.
///
/// The page key will be reused. This will preserve the state and not run any
/// page animation.
///
/// See also:
/// * [push] which pushes the given location onto the page stack.
/// * [pushReplacement] which replaces the top-most page of the page stack but
/// always uses a new page key.
void replace(String location, {Object? extra}) =>
GoRouter.of(this).replace<Object?>(location, extra: extra);
/// Replaces the top-most page with the named route and optional parameters,
/// preserving the page key.
///
/// This will preserve the state and not run any page animation. Optional
/// parameters can be provided to the named route, e.g. `name='person',
/// pathParameters={'fid': 'f2', 'pid': 'p1'}`.
///
/// See also:
/// * [pushNamed] which pushes the given location onto the page stack.
/// * [pushReplacementNamed] which replaces the top-most page of the page
/// stack but always uses a new page key.
void replaceNamed(
String name, {
Map<String, String> pathParameters = const <String, String>{},
Map<String, dynamic> queryParameters = const <String, dynamic>{},
Object? extra,
}) =>
GoRouter.of(this).replaceNamed<Object?>(name,
pathParameters: pathParameters,
queryParameters: queryParameters,
extra: extra);
}
| packages/packages/go_router/lib/src/misc/extensions.dart/0 | {
"file_path": "packages/packages/go_router/lib/src/misc/extensions.dart",
"repo_id": "packages",
"token_count": 1726
} | 973 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:go_router/go_router.dart';
void main() {
testWidgets('CustomTransitionPage builds its child using transitionsBuilder',
(WidgetTester tester) async {
const HomeScreen child = HomeScreen();
final CustomTransitionPage<void> transition = CustomTransitionPage<void>(
transitionsBuilder: expectAsync4((_, __, ___, Widget child) => child),
child: child,
);
final GoRouter router = GoRouter(
routes: <GoRoute>[
GoRoute(
path: '/',
pageBuilder: (_, __) => transition,
),
],
);
addTearDown(router.dispose);
await tester.pumpWidget(
MaterialApp.router(
routerConfig: router,
title: 'GoRouter Example',
),
);
expect(find.byWidget(child), findsOneWidget);
});
testWidgets('NoTransitionPage does not apply any transition',
(WidgetTester tester) async {
final ValueNotifier<bool> showHomeValueNotifier =
ValueNotifier<bool>(false);
addTearDown(showHomeValueNotifier.dispose);
await tester.pumpWidget(
MaterialApp(
home: ValueListenableBuilder<bool>(
valueListenable: showHomeValueNotifier,
builder: (_, bool showHome, __) {
return Navigator(
pages: <Page<void>>[
const NoTransitionPage<void>(
child: LoginScreen(),
),
if (showHome)
const NoTransitionPage<void>(
child: HomeScreen(),
),
],
onPopPage: (Route<dynamic> route, dynamic result) {
return route.didPop(result);
},
);
},
),
),
);
final Finder homeScreenFinder = find.byType(HomeScreen);
expect(homeScreenFinder, findsNothing);
showHomeValueNotifier.value = true;
await tester.pump();
expect(homeScreenFinder, findsOneWidget);
await tester.pumpAndSettle();
showHomeValueNotifier.value = false;
await tester.pump();
expect(homeScreenFinder, findsNothing);
await tester.pumpAndSettle();
});
testWidgets('NoTransitionPage does not apply any reverse transition',
(WidgetTester tester) async {
final ValueNotifier<bool> showHomeValueNotifier = ValueNotifier<bool>(true);
addTearDown(showHomeValueNotifier.dispose);
await tester.pumpWidget(
MaterialApp(
home: ValueListenableBuilder<bool>(
valueListenable: showHomeValueNotifier,
builder: (_, bool showHome, __) {
return Navigator(
pages: <Page<void>>[
const NoTransitionPage<void>(
child: LoginScreen(),
),
if (showHome)
const NoTransitionPage<void>(
child: HomeScreen(),
),
],
onPopPage: (Route<dynamic> route, dynamic result) {
return route.didPop(result);
},
);
},
),
),
);
final Finder homeScreenFinder = find.byType(HomeScreen);
showHomeValueNotifier.value = false;
await tester.pump();
expect(homeScreenFinder, findsNothing);
});
testWidgets('Dismiss a screen by tapping a modal barrier',
(WidgetTester tester) async {
const ValueKey<String> homeKey = ValueKey<String>('home');
const ValueKey<String> dismissibleModalKey =
ValueKey<String>('dismissibleModal');
final GoRouter router = GoRouter(
routes: <GoRoute>[
GoRoute(
path: '/',
builder: (_, __) => const HomeScreen(key: homeKey),
),
GoRoute(
path: '/dismissible-modal',
pageBuilder: (_, GoRouterState state) => CustomTransitionPage<void>(
key: state.pageKey,
barrierDismissible: true,
transitionsBuilder: (_, __, ___, Widget child) => child,
child: const DismissibleModal(key: dismissibleModalKey),
),
),
],
);
addTearDown(router.dispose);
await tester.pumpWidget(MaterialApp.router(routerConfig: router));
expect(find.byKey(homeKey), findsOneWidget);
router.push('/dismissible-modal');
await tester.pumpAndSettle();
expect(find.byKey(dismissibleModalKey), findsOneWidget);
await tester.tapAt(const Offset(50, 50));
await tester.pumpAndSettle();
expect(find.byKey(homeKey), findsOneWidget);
});
testWidgets('transitionDuration and reverseTransitionDuration is different',
(WidgetTester tester) async {
const ValueKey<String> homeKey = ValueKey<String>('home');
const ValueKey<String> loginKey = ValueKey<String>('login');
const Duration transitionDuration = Duration(milliseconds: 50);
const Duration reverseTransitionDuration = Duration(milliseconds: 500);
final GoRouter router = GoRouter(
routes: <GoRoute>[
GoRoute(
path: '/',
builder: (_, __) => const HomeScreen(key: homeKey),
),
GoRoute(
path: '/login',
pageBuilder: (_, GoRouterState state) => CustomTransitionPage<void>(
key: state.pageKey,
transitionDuration: transitionDuration,
reverseTransitionDuration: reverseTransitionDuration,
transitionsBuilder:
(_, Animation<double> animation, ___, Widget child) =>
FadeTransition(opacity: animation, child: child),
child: const LoginScreen(key: loginKey),
),
),
],
);
addTearDown(router.dispose);
await tester.pumpWidget(MaterialApp.router(routerConfig: router));
expect(find.byKey(homeKey), findsOneWidget);
router.push('/login');
final int pushingPumped = await tester.pumpAndSettle();
expect(find.byKey(loginKey), findsOneWidget);
router.pop();
final int poppingPumped = await tester.pumpAndSettle();
expect(find.byKey(homeKey), findsOneWidget);
expect(pushingPumped != poppingPumped, true);
});
}
class HomeScreen extends StatelessWidget {
const HomeScreen({super.key});
@override
Widget build(BuildContext context) {
return const Scaffold(
body: Center(
child: Text('HomeScreen'),
),
);
}
}
class LoginScreen extends StatelessWidget {
const LoginScreen({super.key});
@override
Widget build(BuildContext context) {
return const Scaffold(
body: Center(
child: Text('LoginScreen'),
),
);
}
}
class DismissibleModal extends StatelessWidget {
const DismissibleModal({super.key});
@override
Widget build(BuildContext context) {
return const SizedBox(
width: 200,
height: 200,
child: Center(child: Text('Dismissible Modal')),
);
}
}
| packages/packages/go_router/test/custom_transition_page_test.dart/0 | {
"file_path": "packages/packages/go_router/test/custom_transition_page_test.dart",
"repo_id": "packages",
"token_count": 2993
} | 974 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:go_router/src/pages/material.dart';
import 'helpers/error_screen_helpers.dart';
void main() {
group('isMaterialApp', () {
testWidgets('returns [true] when MaterialApp is present',
(WidgetTester tester) async {
final GlobalKey<_DummyStatefulWidgetState> key =
GlobalKey<_DummyStatefulWidgetState>();
await tester.pumpWidget(
MaterialApp(
home: DummyStatefulWidget(key: key),
),
);
final bool isMaterial = isMaterialApp(key.currentContext! as Element);
expect(isMaterial, true);
});
testWidgets('returns [false] when CupertinoApp is present',
(WidgetTester tester) async {
final GlobalKey<_DummyStatefulWidgetState> key =
GlobalKey<_DummyStatefulWidgetState>();
await tester.pumpWidget(
CupertinoApp(
home: DummyStatefulWidget(key: key),
),
);
final bool isMaterial = isMaterialApp(key.currentContext! as Element);
expect(isMaterial, false);
});
});
test('pageBuilderForMaterialApp creates a [MaterialPage] accordingly', () {
final UniqueKey key = UniqueKey();
const String name = 'name';
const String arguments = 'arguments';
const String restorationId = 'restorationId';
const DummyStatefulWidget child = DummyStatefulWidget();
final MaterialPage<void> page = pageBuilderForMaterialApp(
key: key,
name: name,
arguments: arguments,
restorationId: restorationId,
child: child,
);
expect(page.key, key);
expect(page.name, name);
expect(page.arguments, arguments);
expect(page.restorationId, restorationId);
expect(page.child, child);
});
group('GoRouterMaterialErrorScreen', () {
testWidgets(
'shows "page not found" by default',
testPageNotFound(
widget: const MaterialApp(
home: MaterialErrorScreen(null),
),
),
);
final Exception exception = Exception('Something went wrong!');
testWidgets(
'shows the exception message when provided',
testPageShowsExceptionMessage(
exception: exception,
widget: MaterialApp(
home: MaterialErrorScreen(exception),
),
),
);
testWidgets(
'clicking the TextButton should redirect to /',
testClickingTheButtonRedirectsToRoot(
buttonFinder: find.byType(TextButton),
widget: const MaterialApp(
home: MaterialErrorScreen(null),
),
),
);
});
}
class DummyStatefulWidget extends StatefulWidget {
const DummyStatefulWidget({super.key});
@override
State<DummyStatefulWidget> createState() => _DummyStatefulWidgetState();
}
class _DummyStatefulWidgetState extends State<DummyStatefulWidget> {
@override
Widget build(BuildContext context) => Container();
}
| packages/packages/go_router/test/material_test.dart/0 | {
"file_path": "packages/packages/go_router/test/material_test.dart",
"repo_id": "packages",
"token_count": 1175
} | 975 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// ignore_for_file: public_member_api_docs
import 'dart:math';
import 'package:flutter/foundation.dart';
enum PersonDetails {
hobbies,
favoriteFood,
favoriteSport,
}
enum SportDetails {
volleyball(
imageUrl: '/sportdetails/url/volleyball.jpg',
playerPerTeam: 6,
accessory: null,
hasNet: true,
),
football(
imageUrl: '/sportdetails/url/Football.jpg',
playerPerTeam: 11,
accessory: null,
hasNet: true,
),
tennis(
imageUrl: '/sportdetails/url/tennis.jpg',
playerPerTeam: 2,
accessory: 'Rackets',
hasNet: true,
),
hockey(
imageUrl: '/sportdetails/url/hockey.jpg',
playerPerTeam: 6,
accessory: 'Hockey sticks',
hasNet: true,
),
;
const SportDetails({
required this.accessory,
required this.hasNet,
required this.imageUrl,
required this.playerPerTeam,
});
final String imageUrl;
final int playerPerTeam;
final String? accessory;
final bool hasNet;
}
/// An enum used only in iterables.
enum CookingRecipe {
burger,
pizza,
tacos,
}
/// sample Person class
class Person {
Person({
required this.id,
required this.name,
required this.age,
this.details = const <PersonDetails, String>{},
});
final int id;
final String name;
final int age;
final Map<PersonDetails, String> details;
}
class Family {
Family({required this.id, required this.name, required this.people});
final String id;
final String name;
final List<Person> people;
Person person(int pid) => people.singleWhere(
(Person p) => p.id == pid,
orElse: () => throw Exception('unknown person $pid for family $id'),
);
}
final List<Family> familyData = <Family>[
Family(
id: 'f1',
name: 'Sells',
people: <Person>[
Person(id: 1, name: 'Chris', age: 52, details: <PersonDetails, String>{
PersonDetails.hobbies: 'coding',
PersonDetails.favoriteFood: 'all of the above',
PersonDetails.favoriteSport: 'football?'
}),
Person(id: 2, name: 'John', age: 27),
Person(id: 3, name: 'Tom', age: 26),
],
),
Family(
id: 'f2',
name: 'Addams',
people: <Person>[
Person(id: 1, name: 'Gomez', age: 55),
Person(id: 2, name: 'Morticia', age: 50),
Person(id: 3, name: 'Pugsley', age: 10),
Person(id: 4, name: 'Wednesday', age: 17),
],
),
Family(
id: 'f3',
name: 'Hunting',
people: <Person>[
Person(id: 1, name: 'Mom', age: 54),
Person(id: 2, name: 'Dad', age: 55),
Person(id: 3, name: 'Will', age: 20),
Person(id: 4, name: 'Marky', age: 21),
Person(id: 5, name: 'Ricky', age: 22),
Person(id: 6, name: 'Danny', age: 23),
Person(id: 7, name: 'Terry', age: 24),
Person(id: 8, name: 'Mikey', age: 25),
Person(id: 9, name: 'Davey', age: 26),
Person(id: 10, name: 'Timmy', age: 27),
Person(id: 11, name: 'Tommy', age: 28),
Person(id: 12, name: 'Joey', age: 29),
Person(id: 13, name: 'Robby', age: 30),
Person(id: 14, name: 'Johnny', age: 31),
Person(id: 15, name: 'Brian', age: 32),
],
),
];
Family familyById(String fid) => familyData.family(fid);
extension on List<Family> {
Family family(String fid) => singleWhere(
(Family f) => f.id == fid,
orElse: () => throw Exception('unknown family $fid'),
);
}
class LoginInfo extends ChangeNotifier {
String _userName = '';
String get userName => _userName;
bool get loggedIn => _userName.isNotEmpty;
void login(String userName) {
_userName = userName;
notifyListeners();
}
void logout() {
_userName = '';
notifyListeners();
}
}
class FamilyPerson {
FamilyPerson({required this.family, required this.person});
final Family family;
final Person person;
}
class Repository {
static final Random rnd = Random();
Future<List<Family>> getFamilies() async {
// simulate network delay
await Future<void>.delayed(const Duration(seconds: 1));
// simulate error
// if (rnd.nextBool()) throw Exception('error fetching families');
// return data "fetched over the network"
return familyData;
}
Future<Family> getFamily(String fid) async =>
(await getFamilies()).family(fid);
Future<FamilyPerson> getPerson(String fid, int pid) async {
final Family family = await getFamily(fid);
return FamilyPerson(family: family, person: family.person(pid));
}
}
| packages/packages/go_router_builder/example/lib/shared/data.dart/0 | {
"file_path": "packages/packages/go_router_builder/example/lib/shared/data.dart",
"repo_id": "packages",
"token_count": 1764
} | 976 |
RouteBase get $defaultValueRoute => GoRouteData.$route(
path: '/default-value-route',
factory: $DefaultValueRouteExtension._fromState,
);
extension $DefaultValueRouteExtension on DefaultValueRoute {
static DefaultValueRoute _fromState(GoRouterState state) => DefaultValueRoute(
param:
_$convertMapValue('param', state.uri.queryParameters, int.parse) ??
0,
);
String get location => GoRouteData.$location(
'/default-value-route',
queryParams: {
if (param != 0) 'param': param.toString(),
},
);
void go(BuildContext context) => context.go(location);
Future<T?> push<T>(BuildContext context) => context.push<T>(location);
void pushReplacement(BuildContext context) =>
context.pushReplacement(location);
void replace(BuildContext context) => context.replace(location);
}
T? _$convertMapValue<T>(
String key,
Map<String, String> map,
T Function(String) converter,
) {
final value = map[key];
return value == null ? null : converter(value);
}
| packages/packages/go_router_builder/test_inputs/default_value.dart.expect/0 | {
"file_path": "packages/packages/go_router_builder/test_inputs/default_value.dart.expect",
"repo_id": "packages",
"token_count": 385
} | 977 |
Default value used with a nullable type. Only non-nullable type can have a default value.
| packages/packages/go_router_builder/test_inputs/nullable_default_value.dart.expect/0 | {
"file_path": "packages/packages/go_router_builder/test_inputs/nullable_default_value.dart.expect",
"repo_id": "packages",
"token_count": 22
} | 978 |
RouteBase get $shellRouteBranchData => StatefulShellBranchData.$branch(
initialLocation: ShellRouteBranchData.$initialLocation,
);
| packages/packages/go_router_builder/test_inputs/statefull_shell_branch_data.dart.expect/0 | {
"file_path": "packages/packages/go_router_builder/test_inputs/statefull_shell_branch_data.dart.expect",
"repo_id": "packages",
"token_count": 44
} | 979 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// the following ignore is needed for downgraded analyzer (casts to JSObject).
// ignore_for_file: unnecessary_cast
import 'dart:async';
import 'dart:js_interop';
import 'dart:js_interop_unsafe';
import 'package:flutter_test/flutter_test.dart';
import 'package:google_identity_services_web/id.dart';
import 'package:google_identity_services_web/oauth2.dart';
import 'package:web/web.dart' as web;
/// Function that lets us expect that a JSObject has a [name] property that matches [matcher].
///
/// Use [createExpectConfigValue] to create one of this functions associated with
/// a specific [JSObject].
typedef ExpectConfigValueFn = void Function(String name, Object? matcher);
/// Creates a [ExpectConfigValueFn] for the `config` [JSObject].
ExpectConfigValueFn createExpectConfigValue(JSObject config) {
return (String name, Object? matcher) {
expect(config[name], matcher, reason: name);
};
}
/// A matcher that checks if: value typeof [thing] == true (in JS).
///
/// See: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/typeof
Matcher isAJs(String thing) => isA<JSAny?>()
.having((JSAny? p0) => p0.typeofEquals(thing), 'typeof "$thing"', isTrue);
/// Installs mock-gis.js in the page.
/// Returns a future that completes when the 'load' event of the script fires.
Future<void> installGisMock() {
final Completer<void> completer = Completer<void>();
final web.HTMLScriptElement script =
web.document.createElement('script') as web.HTMLScriptElement;
script.src = 'mock-gis.js';
script.type = 'module';
script.addEventListener(
'load',
(JSAny? _) {
completer.complete();
}.toJS);
web.document.head!.appendChild(script);
return completer.future;
}
/// Fakes authorization with the given scopes.
Future<TokenResponse> fakeAuthZWithScopes(List<String> scopes) {
final StreamController<TokenResponse> controller =
StreamController<TokenResponse>();
final TokenClient client = oauth2.initTokenClient(TokenClientConfig(
client_id: 'for-tests',
callback: controller.add,
scope: scopes,
));
setMockTokenResponse(client, 'some-non-null-auth-token-value');
client.requestAccessToken();
return controller.stream.first;
}
/// Allows calling a `setMockTokenResponse` method (added by mock-gis.js)
extension on TokenClient {
external void setMockTokenResponse(JSString? token);
}
/// Sets a mock TokenResponse value in a [client].
void setMockTokenResponse(TokenClient client, [String? authToken]) {
client.setMockTokenResponse(authToken?.toJS);
}
/// Allows calling a `setMockCredentialResponse` method (set by mock-gis.js)
extension on GoogleAccountsId {
external void setMockCredentialResponse(
JSString credential,
JSString select_by, //ignore: non_constant_identifier_names
);
}
/// Sets a mock credential response in `google.accounts.id`.
void setMockCredentialResponse([String value = 'default_value']) {
_getGoogleAccountsId().setMockCredentialResponse(value.toJS, 'auto'.toJS);
}
GoogleAccountsId _getGoogleAccountsId() {
return _getDeepProperty<GoogleAccountsId>(
web.window as JSObject, 'google.accounts.id');
}
// Attempts to retrieve a deeply nested property from a jsObject (or die tryin')
T _getDeepProperty<T>(JSObject jsObject, String deepProperty) {
final List<String> properties = deepProperty.split('.');
return properties.fold<JSObject?>(
jsObject,
(JSObject? jsObj, String prop) => jsObj?[prop] as JSObject?,
) as T;
}
| packages/packages/google_identity_services_web/example/integration_test/utils.dart/0 | {
"file_path": "packages/packages/google_identity_services_web/example/integration_test/utils.dart",
"repo_id": "packages",
"token_count": 1194
} | 980 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file contains shared definitions used across multiple test scenarios.
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:google_maps_flutter/google_maps_flutter.dart';
/// Initial map center
const LatLng kInitialMapCenter = LatLng(0, 0);
/// Initial zoom level
const double kInitialZoomLevel = 5;
/// Initial camera position
const CameraPosition kInitialCameraPosition =
CameraPosition(target: kInitialMapCenter, zoom: kInitialZoomLevel);
// Dummy map ID
const String kCloudMapId = '000000000000000'; // Dummy map ID.
/// True if the test is running in an iOS device
final bool isIOS = defaultTargetPlatform == TargetPlatform.iOS;
/// True if the test is running in an Android device
final bool isAndroid =
defaultTargetPlatform == TargetPlatform.android && !kIsWeb;
/// True if the test is running in a web browser.
const bool isWeb = kIsWeb;
/// Pumps a [map] widget in [tester] of a certain [size], then waits until it settles.
Future<void> pumpMap(WidgetTester tester, GoogleMap map,
[Size size = const Size.square(200)]) async {
await tester.pumpWidget(wrapMap(map, size));
await tester.pumpAndSettle();
}
/// Wraps a [map] in a bunch of widgets so it renders in all platforms.
///
/// An optional [size] can be passed.
Widget wrapMap(GoogleMap map, [Size size = const Size.square(200)]) {
return MaterialApp(
home: Scaffold(
body: Center(
child: SizedBox.fromSize(
size: size,
child: map,
),
),
),
);
}
| packages/packages/google_maps_flutter/google_maps_flutter/example/integration_test/src/shared.dart/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter/example/integration_test/src/shared.dart",
"repo_id": "packages",
"token_count": 562
} | 981 |
buildscript {
repositories {
google()
mavenCentral()
}
dependencies {
classpath 'com.android.tools.build:gradle:7.4.2'
}
}
allprojects {
repositories {
// See https://github.com/flutter/flutter/wiki/Plugins-and-Packages-repository-structure#gradle-structure for more info.
def artifactRepoKey = 'ARTIFACT_HUB_REPOSITORY'
if (System.getenv().containsKey(artifactRepoKey)) {
println "Using artifact hub"
maven { url System.getenv(artifactRepoKey) }
}
google()
mavenCentral()
}
}
rootProject.buildDir = '../build'
subprojects {
project.buildDir = "${rootProject.buildDir}/${project.name}"
}
subprojects {
project.evaluationDependsOn(':app')
}
tasks.register("clean", Delete) {
delete rootProject.buildDir
}
// Build the plugin project with warnings enabled. This is here rather than
// in the plugin itself to avoid breaking clients that have different
// warnings (e.g., deprecation warnings from a newer SDK than this project
// builds with).
gradle.projectsEvaluated {
project(":google_maps_flutter_android") {
tasks.withType(JavaCompile) {
// Ignore classfile warnings due to https://bugs.openjdk.org/browse/JDK-8190452
// TODO(stuartmorgan): Remove that ignore once the build uses Java 11+.
options.compilerArgs << "-Xlint:all" << "-Werror" << "-Xlint:-classfile"
// Workaround for a warning when building that the above turns into
// an error, coming from jetified-play-services-maps-18.1.0:
// warning: Cannot find annotation method 'value()' in type
// 'GuardedBy': class file for
// javax.annotation.concurrent.GuardedBy not found
dependencies {
implementation "com.google.code.findbugs:jsr305:3.0.2"
}
}
}
}
| packages/packages/google_maps_flutter/google_maps_flutter_android/example/android/build.gradle/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_android/example/android/build.gradle",
"repo_id": "packages",
"token_count": 783
} | 982 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:maps_example_dart/animate_camera.dart';
import 'package:maps_example_dart/lite_mode.dart';
import 'package:maps_example_dart/map_click.dart';
import 'package:maps_example_dart/map_coordinates.dart';
import 'package:maps_example_dart/map_map_id.dart';
import 'package:maps_example_dart/map_ui.dart';
import 'package:maps_example_dart/maps_demo.dart';
import 'package:maps_example_dart/marker_icons.dart';
import 'package:maps_example_dart/move_camera.dart';
import 'package:maps_example_dart/padding.dart';
import 'package:maps_example_dart/page.dart';
import 'package:maps_example_dart/place_circle.dart';
import 'package:maps_example_dart/place_marker.dart';
import 'package:maps_example_dart/place_polygon.dart';
import 'package:maps_example_dart/place_polyline.dart';
import 'package:maps_example_dart/scrolling_map.dart';
import 'package:maps_example_dart/snapshot.dart';
import 'package:maps_example_dart/tile_overlay.dart';
void main() {
runApp(const MaterialApp(
home: MapsDemo(<GoogleMapExampleAppPage>[
MapUiPage(),
MapCoordinatesPage(),
MapClickPage(),
AnimateCameraPage(),
MoveCameraPage(),
PlaceMarkerPage(),
MarkerIconsPage(),
ScrollingMapPage(),
PlacePolylinePage(),
PlacePolygonPage(),
PlaceCirclePage(),
PaddingPage(),
SnapshotPage(),
LiteModePage(),
TileOverlayPage(),
MapIdPage(),
])));
}
| packages/packages/google_maps_flutter/google_maps_flutter_ios/example/ios14/lib/main.dart/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_ios/example/ios14/lib/main.dart",
"repo_id": "packages",
"token_count": 583
} | 983 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_test/flutter_test.dart';
import 'package:google_maps_flutter_platform_interface/google_maps_flutter_platform_interface.dart';
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
group('$Marker', () {
test('constructor defaults', () {
const Marker marker = Marker(markerId: MarkerId('ABC123'));
expect(marker.alpha, equals(1.0));
expect(marker.anchor, equals(const Offset(0.5, 1.0)));
expect(marker.consumeTapEvents, equals(false));
expect(marker.draggable, equals(false));
expect(marker.flat, equals(false));
expect(marker.icon, equals(BitmapDescriptor.defaultMarker));
expect(marker.infoWindow, equals(InfoWindow.noText));
expect(marker.position, equals(const LatLng(0.0, 0.0)));
expect(marker.rotation, equals(0.0));
expect(marker.visible, equals(true));
expect(marker.zIndex, equals(0.0));
expect(marker.onTap, equals(null));
expect(marker.onDrag, equals(null));
expect(marker.onDragStart, equals(null));
expect(marker.onDragEnd, equals(null));
});
test('constructor alpha is >= 0.0 and <= 1.0', () {
void initWithAlpha(double alpha) {
Marker(markerId: const MarkerId('ABC123'), alpha: alpha);
}
expect(() => initWithAlpha(-0.5), throwsAssertionError);
expect(() => initWithAlpha(0.0), isNot(throwsAssertionError));
expect(() => initWithAlpha(0.5), isNot(throwsAssertionError));
expect(() => initWithAlpha(1.0), isNot(throwsAssertionError));
expect(() => initWithAlpha(100), throwsAssertionError);
});
test('toJson', () {
final BitmapDescriptor testDescriptor =
BitmapDescriptor.defaultMarkerWithHue(BitmapDescriptor.hueCyan);
final Marker marker = Marker(
markerId: const MarkerId('ABC123'),
alpha: 0.12345,
anchor: const Offset(100, 100),
consumeTapEvents: true,
draggable: true,
flat: true,
icon: testDescriptor,
infoWindow: const InfoWindow(
title: 'Test title',
snippet: 'Test snippet',
anchor: Offset(100, 200),
),
position: const LatLng(50, 50),
rotation: 100,
visible: false,
zIndex: 100,
onTap: () {},
onDragStart: (LatLng latLng) {},
onDrag: (LatLng latLng) {},
onDragEnd: (LatLng latLng) {},
);
final Map<String, Object> json = marker.toJson() as Map<String, Object>;
expect(json, <String, Object>{
'markerId': 'ABC123',
'alpha': 0.12345,
'anchor': <double>[100, 100],
'consumeTapEvents': true,
'draggable': true,
'flat': true,
'icon': testDescriptor.toJson(),
'infoWindow': <String, Object>{
'title': 'Test title',
'snippet': 'Test snippet',
'anchor': <Object>[100.0, 200.0],
},
'position': <double>[50, 50],
'rotation': 100.0,
'visible': false,
'zIndex': 100.0,
});
});
test('clone', () {
const Marker marker = Marker(markerId: MarkerId('ABC123'));
final Marker clone = marker.clone();
expect(identical(clone, marker), isFalse);
expect(clone, equals(marker));
});
test('copyWith', () {
const MarkerId markerId = MarkerId('ABC123');
const Marker marker = Marker(markerId: markerId);
final BitmapDescriptor testDescriptor =
BitmapDescriptor.defaultMarkerWithHue(BitmapDescriptor.hueCyan);
const double testAlphaParam = 0.12345;
const Offset testAnchorParam = Offset(100, 100);
final bool testConsumeTapEventsParam = !marker.consumeTapEvents;
final bool testDraggableParam = !marker.draggable;
final bool testFlatParam = !marker.flat;
final BitmapDescriptor testIconParam = testDescriptor;
const InfoWindow testInfoWindowParam = InfoWindow(title: 'Test');
const LatLng testPositionParam = LatLng(100, 100);
const double testRotationParam = 100;
final bool testVisibleParam = !marker.visible;
const double testZIndexParam = 100;
const ClusterManagerId testClusterManagerIdParam =
ClusterManagerId('DEF123');
final List<String> log = <String>[];
final Marker copy = marker.copyWith(
alphaParam: testAlphaParam,
anchorParam: testAnchorParam,
consumeTapEventsParam: testConsumeTapEventsParam,
draggableParam: testDraggableParam,
flatParam: testFlatParam,
iconParam: testIconParam,
infoWindowParam: testInfoWindowParam,
positionParam: testPositionParam,
rotationParam: testRotationParam,
visibleParam: testVisibleParam,
zIndexParam: testZIndexParam,
clusterManagerIdParam: testClusterManagerIdParam,
onTapParam: () {
log.add('onTapParam');
},
onDragStartParam: (LatLng latLng) {
log.add('onDragStartParam');
},
onDragParam: (LatLng latLng) {
log.add('onDragParam');
},
onDragEndParam: (LatLng latLng) {
log.add('onDragEndParam');
},
);
expect(copy.markerId, equals(markerId));
expect(copy.alpha, equals(testAlphaParam));
expect(copy.anchor, equals(testAnchorParam));
expect(copy.consumeTapEvents, equals(testConsumeTapEventsParam));
expect(copy.draggable, equals(testDraggableParam));
expect(copy.flat, equals(testFlatParam));
expect(copy.icon, equals(testIconParam));
expect(copy.infoWindow, equals(testInfoWindowParam));
expect(copy.position, equals(testPositionParam));
expect(copy.rotation, equals(testRotationParam));
expect(copy.visible, equals(testVisibleParam));
expect(copy.zIndex, equals(testZIndexParam));
expect(copy.clusterManagerId, equals(testClusterManagerIdParam));
copy.onTap!();
expect(log, contains('onTapParam'));
copy.onDragStart!(const LatLng(0, 1));
expect(log, contains('onDragStartParam'));
copy.onDrag!(const LatLng(0, 1));
expect(log, contains('onDragParam'));
copy.onDragEnd!(const LatLng(0, 1));
expect(log, contains('onDragEndParam'));
});
});
}
| packages/packages/google_maps_flutter/google_maps_flutter_platform_interface/test/types/marker_test.dart/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_platform_interface/test/types/marker_test.dart",
"repo_id": "packages",
"token_count": 2703
} | 984 |
// Mocks generated by Mockito 5.4.4 from annotations
// in google_maps_flutter_web_integration_tests/integration_test/google_maps_plugin_test.dart.
// Do not manually edit this file.
// ignore_for_file: no_leading_underscores_for_library_prefixes
import 'dart:async' as _i3;
import 'package:google_maps/google_maps.dart' as _i5;
import 'package:google_maps_flutter_platform_interface/google_maps_flutter_platform_interface.dart'
as _i2;
import 'package:google_maps_flutter_web/google_maps_flutter_web.dart' as _i4;
import 'package:mockito/mockito.dart' as _i1;
// ignore_for_file: type=lint
// ignore_for_file: avoid_redundant_argument_values
// ignore_for_file: avoid_setters_without_getters
// ignore_for_file: comment_references
// ignore_for_file: deprecated_member_use
// ignore_for_file: deprecated_member_use_from_same_package
// ignore_for_file: implementation_imports
// ignore_for_file: invalid_use_of_visible_for_testing_member
// ignore_for_file: prefer_const_constructors
// ignore_for_file: unnecessary_parenthesis
// ignore_for_file: camel_case_types
// ignore_for_file: subtype_of_sealed_class
class _FakeMapConfiguration_0 extends _i1.SmartFake
implements _i2.MapConfiguration {
_FakeMapConfiguration_0(
Object parent,
Invocation parentInvocation,
) : super(
parent,
parentInvocation,
);
}
class _FakeStreamController_1<T> extends _i1.SmartFake
implements _i3.StreamController<T> {
_FakeStreamController_1(
Object parent,
Invocation parentInvocation,
) : super(
parent,
parentInvocation,
);
}
class _FakeLatLngBounds_2 extends _i1.SmartFake implements _i2.LatLngBounds {
_FakeLatLngBounds_2(
Object parent,
Invocation parentInvocation,
) : super(
parent,
parentInvocation,
);
}
class _FakeScreenCoordinate_3 extends _i1.SmartFake
implements _i2.ScreenCoordinate {
_FakeScreenCoordinate_3(
Object parent,
Invocation parentInvocation,
) : super(
parent,
parentInvocation,
);
}
class _FakeLatLng_4 extends _i1.SmartFake implements _i2.LatLng {
_FakeLatLng_4(
Object parent,
Invocation parentInvocation,
) : super(
parent,
parentInvocation,
);
}
/// A class which mocks [GoogleMapController].
///
/// See the documentation for Mockito's code generation for more information.
class MockGoogleMapController extends _i1.Mock
implements _i4.GoogleMapController {
@override
_i2.MapConfiguration get configuration => (super.noSuchMethod(
Invocation.getter(#configuration),
returnValue: _FakeMapConfiguration_0(
this,
Invocation.getter(#configuration),
),
returnValueForMissingStub: _FakeMapConfiguration_0(
this,
Invocation.getter(#configuration),
),
) as _i2.MapConfiguration);
@override
_i3.StreamController<_i2.MapEvent<Object?>> get stream => (super.noSuchMethod(
Invocation.getter(#stream),
returnValue: _FakeStreamController_1<_i2.MapEvent<Object?>>(
this,
Invocation.getter(#stream),
),
returnValueForMissingStub:
_FakeStreamController_1<_i2.MapEvent<Object?>>(
this,
Invocation.getter(#stream),
),
) as _i3.StreamController<_i2.MapEvent<Object?>>);
@override
_i3.Stream<_i2.MapEvent<Object?>> get events => (super.noSuchMethod(
Invocation.getter(#events),
returnValue: _i3.Stream<_i2.MapEvent<Object?>>.empty(),
returnValueForMissingStub: _i3.Stream<_i2.MapEvent<Object?>>.empty(),
) as _i3.Stream<_i2.MapEvent<Object?>>);
@override
bool get isInitialized => (super.noSuchMethod(
Invocation.getter(#isInitialized),
returnValue: false,
returnValueForMissingStub: false,
) as bool);
@override
List<_i5.MapTypeStyle> get styles => (super.noSuchMethod(
Invocation.getter(#styles),
returnValue: <_i5.MapTypeStyle>[],
returnValueForMissingStub: <_i5.MapTypeStyle>[],
) as List<_i5.MapTypeStyle>);
@override
void debugSetOverrides({
_i4.DebugCreateMapFunction? createMap,
_i4.DebugSetOptionsFunction? setOptions,
_i4.MarkersController? markers,
_i4.CirclesController? circles,
_i4.PolygonsController? polygons,
_i4.PolylinesController? polylines,
_i4.TileOverlaysController? tileOverlays,
}) =>
super.noSuchMethod(
Invocation.method(
#debugSetOverrides,
[],
{
#createMap: createMap,
#setOptions: setOptions,
#markers: markers,
#circles: circles,
#polygons: polygons,
#polylines: polylines,
#tileOverlays: tileOverlays,
},
),
returnValueForMissingStub: null,
);
@override
void init() => super.noSuchMethod(
Invocation.method(
#init,
[],
),
returnValueForMissingStub: null,
);
@override
void updateMapConfiguration(_i2.MapConfiguration? update) =>
super.noSuchMethod(
Invocation.method(
#updateMapConfiguration,
[update],
),
returnValueForMissingStub: null,
);
@override
void updateStyles(List<_i5.MapTypeStyle>? styles) => super.noSuchMethod(
Invocation.method(
#updateStyles,
[styles],
),
returnValueForMissingStub: null,
);
@override
_i3.Future<_i2.LatLngBounds> getVisibleRegion() => (super.noSuchMethod(
Invocation.method(
#getVisibleRegion,
[],
),
returnValue: _i3.Future<_i2.LatLngBounds>.value(_FakeLatLngBounds_2(
this,
Invocation.method(
#getVisibleRegion,
[],
),
)),
returnValueForMissingStub:
_i3.Future<_i2.LatLngBounds>.value(_FakeLatLngBounds_2(
this,
Invocation.method(
#getVisibleRegion,
[],
),
)),
) as _i3.Future<_i2.LatLngBounds>);
@override
_i3.Future<_i2.ScreenCoordinate> getScreenCoordinate(_i2.LatLng? latLng) =>
(super.noSuchMethod(
Invocation.method(
#getScreenCoordinate,
[latLng],
),
returnValue:
_i3.Future<_i2.ScreenCoordinate>.value(_FakeScreenCoordinate_3(
this,
Invocation.method(
#getScreenCoordinate,
[latLng],
),
)),
returnValueForMissingStub:
_i3.Future<_i2.ScreenCoordinate>.value(_FakeScreenCoordinate_3(
this,
Invocation.method(
#getScreenCoordinate,
[latLng],
),
)),
) as _i3.Future<_i2.ScreenCoordinate>);
@override
_i3.Future<_i2.LatLng> getLatLng(_i2.ScreenCoordinate? screenCoordinate) =>
(super.noSuchMethod(
Invocation.method(
#getLatLng,
[screenCoordinate],
),
returnValue: _i3.Future<_i2.LatLng>.value(_FakeLatLng_4(
this,
Invocation.method(
#getLatLng,
[screenCoordinate],
),
)),
returnValueForMissingStub: _i3.Future<_i2.LatLng>.value(_FakeLatLng_4(
this,
Invocation.method(
#getLatLng,
[screenCoordinate],
),
)),
) as _i3.Future<_i2.LatLng>);
@override
_i3.Future<void> moveCamera(_i2.CameraUpdate? cameraUpdate) =>
(super.noSuchMethod(
Invocation.method(
#moveCamera,
[cameraUpdate],
),
returnValue: _i3.Future<void>.value(),
returnValueForMissingStub: _i3.Future<void>.value(),
) as _i3.Future<void>);
@override
_i3.Future<double> getZoomLevel() => (super.noSuchMethod(
Invocation.method(
#getZoomLevel,
[],
),
returnValue: _i3.Future<double>.value(0.0),
returnValueForMissingStub: _i3.Future<double>.value(0.0),
) as _i3.Future<double>);
@override
void updateCircles(_i2.CircleUpdates? updates) => super.noSuchMethod(
Invocation.method(
#updateCircles,
[updates],
),
returnValueForMissingStub: null,
);
@override
void updatePolygons(_i2.PolygonUpdates? updates) => super.noSuchMethod(
Invocation.method(
#updatePolygons,
[updates],
),
returnValueForMissingStub: null,
);
@override
void updatePolylines(_i2.PolylineUpdates? updates) => super.noSuchMethod(
Invocation.method(
#updatePolylines,
[updates],
),
returnValueForMissingStub: null,
);
@override
void updateMarkers(_i2.MarkerUpdates? updates) => super.noSuchMethod(
Invocation.method(
#updateMarkers,
[updates],
),
returnValueForMissingStub: null,
);
@override
void updateTileOverlays(Set<_i2.TileOverlay>? newOverlays) =>
super.noSuchMethod(
Invocation.method(
#updateTileOverlays,
[newOverlays],
),
returnValueForMissingStub: null,
);
@override
void clearTileCache(_i2.TileOverlayId? id) => super.noSuchMethod(
Invocation.method(
#clearTileCache,
[id],
),
returnValueForMissingStub: null,
);
@override
void showInfoWindow(_i2.MarkerId? markerId) => super.noSuchMethod(
Invocation.method(
#showInfoWindow,
[markerId],
),
returnValueForMissingStub: null,
);
@override
void hideInfoWindow(_i2.MarkerId? markerId) => super.noSuchMethod(
Invocation.method(
#hideInfoWindow,
[markerId],
),
returnValueForMissingStub: null,
);
@override
bool isInfoWindowShown(_i2.MarkerId? markerId) => (super.noSuchMethod(
Invocation.method(
#isInfoWindowShown,
[markerId],
),
returnValue: false,
returnValueForMissingStub: false,
) as bool);
@override
void dispose() => super.noSuchMethod(
Invocation.method(
#dispose,
[],
),
returnValueForMissingStub: null,
);
}
| packages/packages/google_maps_flutter/google_maps_flutter_web/example/integration_test/google_maps_plugin_test.mocks.dart/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_web/example/integration_test/google_maps_plugin_test.mocks.dart",
"repo_id": "packages",
"token_count": 4843
} | 985 |
## 5.7.4
* Improves type handling in Objective-C code.
* Updates minimum iOS version to 12.0 and minimum Flutter version to 3.16.6.
## 5.7.3
* Adds privacy manifest.
## 5.7.2
* Updates `clearAuthCache` override to match base class declaration.
## 5.7.1
* Changes `pigeon` to a dev dependency.
## 5.7.0
* Adds support for macOS.
* Updates minimum supported SDK version to Flutter 3.16/Dart 3.2.
## 5.6.5
* Upgrades GoogleSignIn iOS SDK to 7.0.
## 5.6.4
* Converts platform communication to Pigeon.
## 5.6.3
* Adds pub topics to package metadata.
* Updates minimum supported SDK version to Flutter 3.7/Dart 2.19.
## 5.6.2
* Updates functions without a prototype to avoid deprecation warning.
## 5.6.1
* Clarifies explanation of endorsement in README.
* Aligns Dart and Flutter SDK constraints.
## 5.6.0
* Updates minimum Flutter version to 3.3 and iOS 11.
## 5.5.2
* Updates links for the merge of flutter/plugins into flutter/packages.
* Updates minimum Flutter version to 3.0.
## 5.5.1
* Fixes passing `serverClientId` via the channelled `init` call
* Updates minimum Flutter version to 2.10.
## 5.5.0
* Adds override for `GoogleSignInPlatform.initWithParams`.
## 5.4.0
* Adds support for `serverClientId` configuration option.
* Makes `Google-Services.info` file optional.
## 5.3.1
* Suppresses warnings for pre-iOS-13 codepaths.
## 5.3.0
* Supports arm64 iOS simulators by increasing GoogleSignIn dependency to version 6.2.
## 5.2.7
* Fixes library_private_types_in_public_api, sort_child_properties_last and use_key_in_widget_constructors
lint warnings.
## 5.2.6
* Switches to an internal method channel, rather than the default.
## 5.2.5
* Splits from `google_sign_in` as a federated implementation.
| packages/packages/google_sign_in/google_sign_in_ios/CHANGELOG.md/0 | {
"file_path": "packages/packages/google_sign_in/google_sign_in_ios/CHANGELOG.md",
"repo_id": "packages",
"token_count": 589
} | 986 |
name: google_sign_in_platform_interface
description: A common platform interface for the google_sign_in plugin.
repository: https://github.com/flutter/packages/tree/main/packages/google_sign_in/google_sign_in_platform_interface
issue_tracker: https://github.com/flutter/flutter/issues?q=is%3Aissue+is%3Aopen+label%3A%22p%3A+google_sign_in%22
# NOTE: We strongly prefer non-breaking changes, even at the expense of a
# less-clean API. See https://flutter.dev/go/platform-interface-breaking-changes
version: 2.4.5
environment:
sdk: ^3.1.0
flutter: ">=3.13.0"
dependencies:
flutter:
sdk: flutter
plugin_platform_interface: ^2.1.7
dev_dependencies:
flutter_test:
sdk: flutter
mockito: 5.4.4
topics:
- authentication
- google-sign-in
| packages/packages/google_sign_in/google_sign_in_platform_interface/pubspec.yaml/0 | {
"file_path": "packages/packages/google_sign_in/google_sign_in_platform_interface/pubspec.yaml",
"repo_id": "packages",
"token_count": 279
} | 987 |
name: google_sign_in_web
description: Flutter plugin for Google Sign-In, a secure authentication system
for signing in with a Google account on Android, iOS and Web.
repository: https://github.com/flutter/packages/tree/main/packages/google_sign_in/google_sign_in_web
issue_tracker: https://github.com/flutter/flutter/issues?q=is%3Aissue+is%3Aopen+label%3A%22p%3A+google_sign_in%22
version: 0.12.4
environment:
sdk: ^3.3.0
flutter: ">=3.19.0"
flutter:
plugin:
implements: google_sign_in
platforms:
web:
pluginClass: GoogleSignInPlugin
fileName: google_sign_in_web.dart
dependencies:
flutter:
sdk: flutter
flutter_web_plugins:
sdk: flutter
google_identity_services_web: ^0.3.1
google_sign_in_platform_interface: ^2.4.0
http: ">=0.13.0 <2.0.0"
web: ^0.5.0
dev_dependencies:
flutter_test:
sdk: flutter
topics:
- authentication
- google-sign-in
| packages/packages/google_sign_in/google_sign_in_web/pubspec.yaml/0 | {
"file_path": "packages/packages/google_sign_in/google_sign_in_web/pubspec.yaml",
"repo_id": "packages",
"token_count": 377
} | 988 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.imagepicker;
import android.content.Context;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.util.Log;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.annotation.VisibleForTesting;
import androidx.core.util.SizeFCompat;
import androidx.exifinterface.media.ExifInterface;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
class ImageResizer {
private final Context context;
private final ExifDataCopier exifDataCopier;
ImageResizer(final @NonNull Context context, final @NonNull ExifDataCopier exifDataCopier) {
this.context = context;
this.exifDataCopier = exifDataCopier;
}
/**
* If necessary, resizes the image located in imagePath and then returns the path for the scaled
* image.
*
* <p>If no resizing is needed, returns the path for the original image.
*/
String resizeImageIfNeeded(
String imagePath, @Nullable Double maxWidth, @Nullable Double maxHeight, int imageQuality) {
SizeFCompat originalSize = readFileDimensions(imagePath);
if (originalSize.getWidth() == -1 || originalSize.getHeight() == -1) {
return imagePath;
}
boolean shouldScale = maxWidth != null || maxHeight != null || imageQuality < 100;
if (!shouldScale) {
return imagePath;
}
try {
String[] pathParts = imagePath.split("/");
String imageName = pathParts[pathParts.length - 1];
SizeFCompat targetSize =
calculateTargetSize(
(double) originalSize.getWidth(),
(double) originalSize.getHeight(),
maxWidth,
maxHeight);
BitmapFactory.Options options = new BitmapFactory.Options();
options.inSampleSize =
calculateSampleSize(options, (int) targetSize.getWidth(), (int) targetSize.getHeight());
Bitmap bmp = decodeFile(imagePath, options);
if (bmp == null) {
return imagePath;
}
File file =
resizedImage(
bmp,
(double) targetSize.getWidth(),
(double) targetSize.getHeight(),
imageQuality,
imageName);
copyExif(imagePath, file.getPath());
return file.getPath();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
private File resizedImage(
Bitmap bmp, Double width, Double height, int imageQuality, String outputImageName)
throws IOException {
Bitmap scaledBmp = createScaledBitmap(bmp, width.intValue(), height.intValue(), false);
File file =
createImageOnExternalDirectory("/scaled_" + outputImageName, scaledBmp, imageQuality);
return file;
}
private SizeFCompat calculateTargetSize(
double originalWidth,
double originalHeight,
@Nullable Double maxWidth,
@Nullable Double maxHeight) {
double aspectRatio = originalWidth / originalHeight;
boolean hasMaxWidth = maxWidth != null;
boolean hasMaxHeight = maxHeight != null;
double width = hasMaxWidth ? Math.min(originalWidth, Math.round(maxWidth)) : originalWidth;
double height = hasMaxHeight ? Math.min(originalHeight, Math.round(maxHeight)) : originalHeight;
boolean shouldDownscaleWidth = hasMaxWidth && maxWidth < originalWidth;
boolean shouldDownscaleHeight = hasMaxHeight && maxHeight < originalHeight;
boolean shouldDownscale = shouldDownscaleWidth || shouldDownscaleHeight;
if (shouldDownscale) {
double WidthForMaxHeight = height * aspectRatio;
double heightForMaxWidth = width / aspectRatio;
if (heightForMaxWidth > height) {
width = (double) Math.round(WidthForMaxHeight);
} else {
height = (double) Math.round(heightForMaxWidth);
}
}
return new SizeFCompat((float) width, (float) height);
}
private File createFile(File externalFilesDirectory, String child) {
File image = new File(externalFilesDirectory, child);
if (!image.getParentFile().exists()) {
image.getParentFile().mkdirs();
}
return image;
}
private FileOutputStream createOutputStream(File imageFile) throws IOException {
return new FileOutputStream(imageFile);
}
private void copyExif(String filePathOri, String filePathDest) {
try {
exifDataCopier.copyExif(new ExifInterface(filePathOri), new ExifInterface(filePathDest));
} catch (Exception ex) {
Log.e("ImageResizer", "Error preserving Exif data on selected image: " + ex);
}
}
@VisibleForTesting
SizeFCompat readFileDimensions(String path) {
BitmapFactory.Options options = new BitmapFactory.Options();
options.inJustDecodeBounds = true;
decodeFile(path, options);
return new SizeFCompat(options.outWidth, options.outHeight);
}
private Bitmap decodeFile(String path, @Nullable BitmapFactory.Options opts) {
return BitmapFactory.decodeFile(path, opts);
}
private Bitmap createScaledBitmap(Bitmap bmp, int width, int height, boolean filter) {
return Bitmap.createScaledBitmap(bmp, width, height, filter);
}
/**
* Calculates the largest sample size value that is a power of two based on a target width and
* height.
*
* <p>This value is necessary to tell the Bitmap decoder to subsample the original image,
* returning a smaller image to save memory.
*
* @see <a
* href="https://developer.android.com/topic/performance/graphics/load-bitmap#load-bitmap">
* Loading Large Bitmaps Efficiently</a>
*/
private int calculateSampleSize(
BitmapFactory.Options options, int targetWidth, int targetHeight) {
final int height = options.outHeight;
final int width = options.outWidth;
int sampleSize = 1;
if (height > targetHeight || width > targetWidth) {
final int halfHeight = height / 2;
final int halfWidth = width / 2;
while ((halfHeight / sampleSize) >= targetHeight && (halfWidth / sampleSize) >= targetWidth) {
sampleSize *= 2;
}
}
return sampleSize;
}
private File createImageOnExternalDirectory(String name, Bitmap bitmap, int imageQuality)
throws IOException {
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
boolean saveAsPNG = bitmap.hasAlpha();
if (saveAsPNG) {
Log.d(
"ImageResizer",
"image_picker: compressing is not supported for type PNG. Returning the image with original quality");
}
bitmap.compress(
saveAsPNG ? Bitmap.CompressFormat.PNG : Bitmap.CompressFormat.JPEG,
imageQuality,
outputStream);
File cacheDirectory = context.getCacheDir();
File imageFile = createFile(cacheDirectory, name);
FileOutputStream fileOutput = createOutputStream(imageFile);
fileOutput.write(outputStream.toByteArray());
fileOutput.close();
return imageFile;
}
}
| packages/packages/image_picker/image_picker_android/android/src/main/java/io/flutter/plugins/imagepicker/ImageResizer.java/0 | {
"file_path": "packages/packages/image_picker/image_picker_android/android/src/main/java/io/flutter/plugins/imagepicker/ImageResizer.java",
"repo_id": "packages",
"token_count": 2456
} | 989 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// Autogenerated from Pigeon (v9.2.5), do not edit directly.
// See also: https://pub.dev/packages/pigeon
// ignore_for_file: public_member_api_docs, non_constant_identifier_names, avoid_as, unused_import, unnecessary_parenthesis, unnecessary_import
// ignore_for_file: avoid_relative_lib_imports
import 'dart:async';
import 'dart:typed_data' show Float64List, Int32List, Int64List, Uint8List;
import 'package:flutter/foundation.dart' show ReadBuffer, WriteBuffer;
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:image_picker_android/src/messages.g.dart';
class _TestHostImagePickerApiCodec extends StandardMessageCodec {
const _TestHostImagePickerApiCodec();
@override
void writeValue(WriteBuffer buffer, Object? value) {
if (value is CacheRetrievalError) {
buffer.putUint8(128);
writeValue(buffer, value.encode());
} else if (value is CacheRetrievalResult) {
buffer.putUint8(129);
writeValue(buffer, value.encode());
} else if (value is GeneralOptions) {
buffer.putUint8(130);
writeValue(buffer, value.encode());
} else if (value is ImageSelectionOptions) {
buffer.putUint8(131);
writeValue(buffer, value.encode());
} else if (value is MediaSelectionOptions) {
buffer.putUint8(132);
writeValue(buffer, value.encode());
} else if (value is SourceSpecification) {
buffer.putUint8(133);
writeValue(buffer, value.encode());
} else if (value is VideoSelectionOptions) {
buffer.putUint8(134);
writeValue(buffer, value.encode());
} else {
super.writeValue(buffer, value);
}
}
@override
Object? readValueOfType(int type, ReadBuffer buffer) {
switch (type) {
case 128:
return CacheRetrievalError.decode(readValue(buffer)!);
case 129:
return CacheRetrievalResult.decode(readValue(buffer)!);
case 130:
return GeneralOptions.decode(readValue(buffer)!);
case 131:
return ImageSelectionOptions.decode(readValue(buffer)!);
case 132:
return MediaSelectionOptions.decode(readValue(buffer)!);
case 133:
return SourceSpecification.decode(readValue(buffer)!);
case 134:
return VideoSelectionOptions.decode(readValue(buffer)!);
default:
return super.readValueOfType(type, buffer);
}
}
}
abstract class TestHostImagePickerApi {
static TestDefaultBinaryMessengerBinding? get _testBinaryMessengerBinding =>
TestDefaultBinaryMessengerBinding.instance;
static const MessageCodec<Object?> codec = _TestHostImagePickerApiCodec();
/// Selects images and returns their paths.
///
/// Elements must not be null, by convention. See
/// https://github.com/flutter/flutter/issues/97848
Future<List<String?>> pickImages(SourceSpecification source,
ImageSelectionOptions options, GeneralOptions generalOptions);
/// Selects video and returns their paths.
///
/// Elements must not be null, by convention. See
/// https://github.com/flutter/flutter/issues/97848
Future<List<String?>> pickVideos(SourceSpecification source,
VideoSelectionOptions options, GeneralOptions generalOptions);
/// Selects images and videos and returns their paths.
///
/// Elements must not be null, by convention. See
/// https://github.com/flutter/flutter/issues/97848
Future<List<String?>> pickMedia(MediaSelectionOptions mediaSelectionOptions,
GeneralOptions generalOptions);
/// Returns results from a previous app session, if any.
CacheRetrievalResult? retrieveLostResults();
static void setup(TestHostImagePickerApi? api,
{BinaryMessenger? binaryMessenger}) {
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ImagePickerApi.pickImages', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
_testBinaryMessengerBinding!.defaultBinaryMessenger
.setMockDecodedMessageHandler<Object?>(channel, null);
} else {
_testBinaryMessengerBinding!.defaultBinaryMessenger
.setMockDecodedMessageHandler<Object?>(channel,
(Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.ImagePickerApi.pickImages was null.');
final List<Object?> args = (message as List<Object?>?)!;
final SourceSpecification? arg_source =
(args[0] as SourceSpecification?);
assert(arg_source != null,
'Argument for dev.flutter.pigeon.ImagePickerApi.pickImages was null, expected non-null SourceSpecification.');
final ImageSelectionOptions? arg_options =
(args[1] as ImageSelectionOptions?);
assert(arg_options != null,
'Argument for dev.flutter.pigeon.ImagePickerApi.pickImages was null, expected non-null ImageSelectionOptions.');
final GeneralOptions? arg_generalOptions =
(args[2] as GeneralOptions?);
assert(arg_generalOptions != null,
'Argument for dev.flutter.pigeon.ImagePickerApi.pickImages was null, expected non-null GeneralOptions.');
final List<String?> output = await api.pickImages(
arg_source!, arg_options!, arg_generalOptions!);
return <Object?>[output];
});
}
}
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ImagePickerApi.pickVideos', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
_testBinaryMessengerBinding!.defaultBinaryMessenger
.setMockDecodedMessageHandler<Object?>(channel, null);
} else {
_testBinaryMessengerBinding!.defaultBinaryMessenger
.setMockDecodedMessageHandler<Object?>(channel,
(Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.ImagePickerApi.pickVideos was null.');
final List<Object?> args = (message as List<Object?>?)!;
final SourceSpecification? arg_source =
(args[0] as SourceSpecification?);
assert(arg_source != null,
'Argument for dev.flutter.pigeon.ImagePickerApi.pickVideos was null, expected non-null SourceSpecification.');
final VideoSelectionOptions? arg_options =
(args[1] as VideoSelectionOptions?);
assert(arg_options != null,
'Argument for dev.flutter.pigeon.ImagePickerApi.pickVideos was null, expected non-null VideoSelectionOptions.');
final GeneralOptions? arg_generalOptions =
(args[2] as GeneralOptions?);
assert(arg_generalOptions != null,
'Argument for dev.flutter.pigeon.ImagePickerApi.pickVideos was null, expected non-null GeneralOptions.');
final List<String?> output = await api.pickVideos(
arg_source!, arg_options!, arg_generalOptions!);
return <Object?>[output];
});
}
}
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ImagePickerApi.pickMedia', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
_testBinaryMessengerBinding!.defaultBinaryMessenger
.setMockDecodedMessageHandler<Object?>(channel, null);
} else {
_testBinaryMessengerBinding!.defaultBinaryMessenger
.setMockDecodedMessageHandler<Object?>(channel,
(Object? message) async {
assert(message != null,
'Argument for dev.flutter.pigeon.ImagePickerApi.pickMedia was null.');
final List<Object?> args = (message as List<Object?>?)!;
final MediaSelectionOptions? arg_mediaSelectionOptions =
(args[0] as MediaSelectionOptions?);
assert(arg_mediaSelectionOptions != null,
'Argument for dev.flutter.pigeon.ImagePickerApi.pickMedia was null, expected non-null MediaSelectionOptions.');
final GeneralOptions? arg_generalOptions =
(args[1] as GeneralOptions?);
assert(arg_generalOptions != null,
'Argument for dev.flutter.pigeon.ImagePickerApi.pickMedia was null, expected non-null GeneralOptions.');
final List<String?> output = await api.pickMedia(
arg_mediaSelectionOptions!, arg_generalOptions!);
return <Object?>[output];
});
}
}
{
final BasicMessageChannel<Object?> channel = BasicMessageChannel<Object?>(
'dev.flutter.pigeon.ImagePickerApi.retrieveLostResults', codec,
binaryMessenger: binaryMessenger);
if (api == null) {
_testBinaryMessengerBinding!.defaultBinaryMessenger
.setMockDecodedMessageHandler<Object?>(channel, null);
} else {
_testBinaryMessengerBinding!.defaultBinaryMessenger
.setMockDecodedMessageHandler<Object?>(channel,
(Object? message) async {
// ignore message
final CacheRetrievalResult? output = api.retrieveLostResults();
return <Object?>[output];
});
}
}
}
}
| packages/packages/image_picker/image_picker_android/test/test_api.g.dart/0 | {
"file_path": "packages/packages/image_picker/image_picker_android/test/test_api.g.dart",
"repo_id": "packages",
"token_count": 3702
} | 990 |
name: image_picker_for_web
description: Web platform implementation of image_picker
repository: https://github.com/flutter/packages/tree/main/packages/image_picker/image_picker_for_web
issue_tracker: https://github.com/flutter/flutter/issues?q=is%3Aissue+is%3Aopen+label%3A%22p%3A+image_picker%22
version: 3.0.3
environment:
sdk: ^3.3.0
flutter: ">=3.19.0"
flutter:
plugin:
implements: image_picker
platforms:
web:
pluginClass: ImagePickerPlugin
fileName: image_picker_for_web.dart
dependencies:
flutter:
sdk: flutter
flutter_web_plugins:
sdk: flutter
image_picker_platform_interface: ^2.9.0
mime: ^1.0.4
web: ^0.5.1
dev_dependencies:
flutter_test:
sdk: flutter
topics:
- camera
- image-picker
- files
- file-selection
| packages/packages/image_picker/image_picker_for_web/pubspec.yaml/0 | {
"file_path": "packages/packages/image_picker/image_picker_for_web/pubspec.yaml",
"repo_id": "packages",
"token_count": 339
} | 991 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#import "ImagePickerTestImages.h"
@import image_picker_ios;
@import image_picker_ios.Test;
@import XCTest;
@interface MetaDataUtilTests : XCTestCase
@end
@implementation MetaDataUtilTests
- (void)testGetImageMIMETypeFromImageData {
// test jpeg
XCTAssertEqual(
[FLTImagePickerMetaDataUtil getImageMIMETypeFromImageData:ImagePickerTestImages.JPGTestData],
FLTImagePickerMIMETypeJPEG);
// test png
XCTAssertEqual(
[FLTImagePickerMetaDataUtil getImageMIMETypeFromImageData:ImagePickerTestImages.PNGTestData],
FLTImagePickerMIMETypePNG);
// test gif
XCTAssertEqual(
[FLTImagePickerMetaDataUtil getImageMIMETypeFromImageData:ImagePickerTestImages.GIFTestData],
FLTImagePickerMIMETypeGIF);
}
- (void)testSuffixFromType {
// test jpeg
XCTAssertEqualObjects(
[FLTImagePickerMetaDataUtil imageTypeSuffixFromType:FLTImagePickerMIMETypeJPEG], @".jpg");
// test png
XCTAssertEqualObjects(
[FLTImagePickerMetaDataUtil imageTypeSuffixFromType:FLTImagePickerMIMETypePNG], @".png");
// test gif
XCTAssertEqualObjects(
[FLTImagePickerMetaDataUtil imageTypeSuffixFromType:FLTImagePickerMIMETypeGIF], @".gif");
// test other
XCTAssertNil([FLTImagePickerMetaDataUtil imageTypeSuffixFromType:FLTImagePickerMIMETypeOther]);
}
- (void)testGetMetaData {
NSDictionary *metaData =
[FLTImagePickerMetaDataUtil getMetaDataFromImageData:ImagePickerTestImages.JPGTestData];
NSDictionary *exif = [metaData objectForKey:(__bridge NSString *)kCGImagePropertyExifDictionary];
XCTAssertEqual([exif[(__bridge NSString *)kCGImagePropertyExifPixelXDimension] integerValue], 12);
}
- (void)testWriteMetaData {
NSData *dataJPG = ImagePickerTestImages.JPGTestData;
NSDictionary *metaData = [FLTImagePickerMetaDataUtil getMetaDataFromImageData:dataJPG];
NSString *tmpFile = [NSString stringWithFormat:@"image_picker_test.jpg"];
NSString *tmpDirectory = NSTemporaryDirectory();
NSString *tmpPath = [tmpDirectory stringByAppendingPathComponent:tmpFile];
NSData *newData = [FLTImagePickerMetaDataUtil imageFromImage:dataJPG withMetaData:metaData];
if ([[NSFileManager defaultManager] createFileAtPath:tmpPath contents:newData attributes:nil]) {
NSData *savedTmpImageData = [NSData dataWithContentsOfFile:tmpPath];
NSDictionary *tmpMetaData =
[FLTImagePickerMetaDataUtil getMetaDataFromImageData:savedTmpImageData];
XCTAssert([tmpMetaData isEqualToDictionary:metaData]);
} else {
XCTAssert(NO);
}
}
- (void)testUpdateMetaDataBadData {
NSData *imageData = [NSData data];
NSDictionary *metaData = [FLTImagePickerMetaDataUtil getMetaDataFromImageData:imageData];
NSData *newData = [FLTImagePickerMetaDataUtil imageFromImage:imageData withMetaData:metaData];
XCTAssertNil(newData);
}
- (void)testConvertImageToData {
UIImage *imageJPG = [UIImage imageWithData:ImagePickerTestImages.JPGTestData];
NSData *convertedDataJPG = [FLTImagePickerMetaDataUtil convertImage:imageJPG
usingType:FLTImagePickerMIMETypeJPEG
quality:@(0.5)];
XCTAssertEqual([FLTImagePickerMetaDataUtil getImageMIMETypeFromImageData:convertedDataJPG],
FLTImagePickerMIMETypeJPEG);
NSData *convertedDataPNG = [FLTImagePickerMetaDataUtil convertImage:imageJPG
usingType:FLTImagePickerMIMETypePNG
quality:nil];
XCTAssertEqual([FLTImagePickerMetaDataUtil getImageMIMETypeFromImageData:convertedDataPNG],
FLTImagePickerMIMETypePNG);
}
@end
| packages/packages/image_picker/image_picker_ios/example/ios/RunnerTests/MetaDataUtilTests.m/0 | {
"file_path": "packages/packages/image_picker/image_picker_ios/example/ios/RunnerTests/MetaDataUtilTests.m",
"repo_id": "packages",
"token_count": 1593
} | 992 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#import <Foundation/Foundation.h>
#import <PhotosUI/PhotosUI.h>
#import "FLTImagePickerImageUtil.h"
#import "FLTImagePickerMetaDataUtil.h"
#import "FLTImagePickerPhotoAssetUtil.h"
NS_ASSUME_NONNULL_BEGIN
/// Returns either the saved path, or an error. Both cannot be set.
typedef void (^FLTGetSavedPath)(NSString *_Nullable savedPath, FlutterError *_Nullable error);
/// @class FLTPHPickerSaveImageToPathOperation
///
/// @brief The FLTPHPickerSaveImageToPathOperation class
///
/// @discussion This class was implemented to handle saved image paths and populate the pathList
/// with the final result by using GetSavedPath type block.
///
/// @superclass SuperClass: NSOperation\n
/// @helps It helps FLTImagePickerPlugin class.
@interface FLTPHPickerSaveImageToPathOperation : NSOperation
- (instancetype)initWithResult:(PHPickerResult *)result
maxHeight:(NSNumber *)maxHeight
maxWidth:(NSNumber *)maxWidth
desiredImageQuality:(NSNumber *)desiredImageQuality
fullMetadata:(BOOL)fullMetadata
savedPathBlock:(FLTGetSavedPath)savedPathBlock API_AVAILABLE(ios(14));
@end
NS_ASSUME_NONNULL_END
| packages/packages/image_picker/image_picker_ios/ios/Classes/FLTPHPickerSaveImageToPathOperation.h/0 | {
"file_path": "packages/packages/image_picker/image_picker_ios/ios/Classes/FLTPHPickerSaveImageToPathOperation.h",
"repo_id": "packages",
"token_count": 477
} | 993 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'types.dart';
/// Specifies options for picking a single image from the device's camera or gallery.
///
/// This class inheritance is a byproduct of the api changing over time.
/// It exists solely to avoid breaking changes.
class ImagePickerOptions extends ImageOptions {
/// Creates an instance with the given [maxHeight], [maxWidth], [imageQuality],
/// [referredCameraDevice] and [requestFullMetadata].
const ImagePickerOptions({
super.maxHeight,
super.maxWidth,
super.imageQuality,
super.requestFullMetadata,
this.preferredCameraDevice = CameraDevice.rear,
}) : super();
/// Creates an instance with the given [maxHeight], [maxWidth], [imageQuality],
/// [referredCameraDevice] and [requestFullMetadata].
ImagePickerOptions.createAndValidate({
super.maxHeight,
super.maxWidth,
super.imageQuality,
super.requestFullMetadata,
this.preferredCameraDevice = CameraDevice.rear,
}) : super.createAndValidate();
/// Used to specify the camera to use when the `source` is [ImageSource.camera].
///
/// Ignored if the source is not [ImageSource.camera], or the chosen camera is not
/// supported on the device. Defaults to [CameraDevice.rear].
final CameraDevice preferredCameraDevice;
}
/// Specifies image-specific options for picking.
class ImageOptions {
/// Creates an instance with the given [maxHeight], [maxWidth], [imageQuality]
/// and [requestFullMetadata].
const ImageOptions({
this.maxHeight,
this.maxWidth,
this.imageQuality,
this.requestFullMetadata = true,
});
/// Creates an instance with the given [maxHeight], [maxWidth], [imageQuality]
/// and [requestFullMetadata]. Throws if options are not valid.
ImageOptions.createAndValidate({
this.maxHeight,
this.maxWidth,
this.imageQuality,
this.requestFullMetadata = true,
}) {
_validateOptions(
maxWidth: maxWidth, maxHeight: maxHeight, imageQuality: imageQuality);
}
/// The maximum width of the image, in pixels.
///
/// If null, the image will only be resized if [maxHeight] is specified.
final double? maxWidth;
/// The maximum height of the image, in pixels.
///
/// If null, the image will only be resized if [maxWidth] is specified.
final double? maxHeight;
/// Modifies the quality of the image, ranging from 0-100 where 100 is the
/// original/max quality.
///
/// Compression is only supported for certain image types such as JPEG. If
/// compression is not supported for the image that is picked, a warning
/// message will be logged.
///
/// If null, the image will be returned with the original quality.
final int? imageQuality;
/// If true, requests full image metadata, which may require extra permissions
/// on some platforms, (e.g., NSPhotoLibraryUsageDescription on iOS).
//
// Defaults to true.
final bool requestFullMetadata;
/// Validates that all values are within required ranges. Throws if not.
static void _validateOptions(
{double? maxWidth, final double? maxHeight, int? imageQuality}) {
if (imageQuality != null && (imageQuality < 0 || imageQuality > 100)) {
throw ArgumentError.value(
imageQuality, 'imageQuality', 'must be between 0 and 100');
}
if (maxWidth != null && maxWidth < 0) {
throw ArgumentError.value(maxWidth, 'maxWidth', 'cannot be negative');
}
if (maxHeight != null && maxHeight < 0) {
throw ArgumentError.value(maxHeight, 'maxHeight', 'cannot be negative');
}
}
}
| packages/packages/image_picker/image_picker_platform_interface/lib/src/types/image_options.dart/0 | {
"file_path": "packages/packages/image_picker/image_picker_platform_interface/lib/src/types/image_options.dart",
"repo_id": "packages",
"token_count": 1096
} | 994 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_test/flutter_test.dart';
import 'package:image_picker_platform_interface/image_picker_platform_interface.dart';
void main() {
group('ImagePickerPlatform', () {
test('supportsImageSource defaults to true for original values', () async {
final ImagePickerPlatform implementation = FakeImagePickerPlatform();
expect(implementation.supportsImageSource(ImageSource.camera), true);
expect(implementation.supportsImageSource(ImageSource.gallery), true);
});
});
group('CameraDelegatingImagePickerPlatform', () {
test(
'supportsImageSource returns false for camera when there is no delegate',
() async {
final FakeCameraDelegatingImagePickerPlatform implementation =
FakeCameraDelegatingImagePickerPlatform();
expect(implementation.supportsImageSource(ImageSource.camera), false);
});
test('supportsImageSource returns true for camera when there is a delegate',
() async {
final FakeCameraDelegatingImagePickerPlatform implementation =
FakeCameraDelegatingImagePickerPlatform();
implementation.cameraDelegate = FakeCameraDelegate();
expect(implementation.supportsImageSource(ImageSource.camera), true);
});
test('getImageFromSource for camera throws if delegate is not set',
() async {
final FakeCameraDelegatingImagePickerPlatform implementation =
FakeCameraDelegatingImagePickerPlatform();
await expectLater(
implementation.getImageFromSource(source: ImageSource.camera),
throwsStateError);
});
test('getVideo for camera throws if delegate is not set', () async {
final FakeCameraDelegatingImagePickerPlatform implementation =
FakeCameraDelegatingImagePickerPlatform();
await expectLater(implementation.getVideo(source: ImageSource.camera),
throwsStateError);
});
test('getImageFromSource for camera calls delegate if set', () async {
const String fakePath = '/tmp/foo';
final FakeCameraDelegatingImagePickerPlatform implementation =
FakeCameraDelegatingImagePickerPlatform();
implementation.cameraDelegate =
FakeCameraDelegate(result: XFile(fakePath));
expect(
(await implementation.getImageFromSource(source: ImageSource.camera))!
.path,
fakePath);
});
test('getVideo for camera calls delegate if set', () async {
const String fakePath = '/tmp/foo';
final FakeCameraDelegatingImagePickerPlatform implementation =
FakeCameraDelegatingImagePickerPlatform();
implementation.cameraDelegate =
FakeCameraDelegate(result: XFile(fakePath));
expect((await implementation.getVideo(source: ImageSource.camera))!.path,
fakePath);
});
});
}
class FakeImagePickerPlatform extends ImagePickerPlatform {}
class FakeCameraDelegatingImagePickerPlatform
extends CameraDelegatingImagePickerPlatform {}
class FakeCameraDelegate extends ImagePickerCameraDelegate {
FakeCameraDelegate({this.result});
XFile? result;
@override
Future<XFile?> takePhoto(
{ImagePickerCameraDelegateOptions options =
const ImagePickerCameraDelegateOptions()}) async {
return result;
}
@override
Future<XFile?> takeVideo(
{ImagePickerCameraDelegateOptions options =
const ImagePickerCameraDelegateOptions()}) async {
return result;
}
}
| packages/packages/image_picker/image_picker_platform_interface/test/image_picker_platform_test.dart/0 | {
"file_path": "packages/packages/image_picker/image_picker_platform_interface/test/image_picker_platform_test.dart",
"repo_id": "packages",
"token_count": 1190
} | 995 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.inapppurchase;
import android.app.Application;
import android.content.Context;
import androidx.annotation.NonNull;
import androidx.annotation.VisibleForTesting;
import com.android.billingclient.api.BillingClient;
import io.flutter.embedding.engine.plugins.FlutterPlugin;
import io.flutter.embedding.engine.plugins.activity.ActivityAware;
import io.flutter.embedding.engine.plugins.activity.ActivityPluginBinding;
import io.flutter.plugin.common.BinaryMessenger;
import io.flutter.plugin.common.MethodChannel;
/** Wraps a {@link BillingClient} instance and responds to Dart calls for it. */
public class InAppPurchasePlugin implements FlutterPlugin, ActivityAware {
static final String PROXY_PACKAGE_KEY = "PROXY_PACKAGE";
// The proxy value has to match the <package> value in library's AndroidManifest.xml.
// This is important that the <package> is not changed, so we hard code the value here then having
// a unit test to make sure. If there is a strong reason to change the <package> value, please inform the
// code owner of this package.
static final String PROXY_VALUE = "io.flutter.plugins.inapppurchase";
private MethodChannel methodChannel;
private MethodCallHandlerImpl methodCallHandler;
/** Plugin registration. */
@SuppressWarnings("deprecation")
public static void registerWith(
@NonNull io.flutter.plugin.common.PluginRegistry.Registrar registrar) {
InAppPurchasePlugin plugin = new InAppPurchasePlugin();
registrar.activity().getIntent().putExtra(PROXY_PACKAGE_KEY, PROXY_VALUE);
((Application) registrar.context().getApplicationContext())
.registerActivityLifecycleCallbacks(plugin.methodCallHandler);
}
@Override
public void onAttachedToEngine(@NonNull FlutterPlugin.FlutterPluginBinding binding) {
setUpMethodChannel(binding.getBinaryMessenger(), binding.getApplicationContext());
}
@Override
public void onDetachedFromEngine(@NonNull FlutterPlugin.FlutterPluginBinding binding) {
teardownMethodChannel();
}
@Override
public void onAttachedToActivity(@NonNull ActivityPluginBinding binding) {
binding.getActivity().getIntent().putExtra(PROXY_PACKAGE_KEY, PROXY_VALUE);
methodCallHandler.setActivity(binding.getActivity());
}
@Override
public void onDetachedFromActivity() {
methodCallHandler.setActivity(null);
methodCallHandler.onDetachedFromActivity();
}
@Override
public void onReattachedToActivityForConfigChanges(@NonNull ActivityPluginBinding binding) {
onAttachedToActivity(binding);
}
@Override
public void onDetachedFromActivityForConfigChanges() {
methodCallHandler.setActivity(null);
}
private void setUpMethodChannel(BinaryMessenger messenger, Context context) {
methodChannel = new MethodChannel(messenger, "plugins.flutter.io/in_app_purchase");
methodCallHandler =
new MethodCallHandlerImpl(
/*activity=*/ null, context, methodChannel, new BillingClientFactoryImpl());
methodChannel.setMethodCallHandler(methodCallHandler);
}
private void teardownMethodChannel() {
methodChannel.setMethodCallHandler(null);
methodChannel = null;
methodCallHandler = null;
}
@VisibleForTesting
void setMethodCallHandler(MethodCallHandlerImpl methodCallHandler) {
this.methodCallHandler = methodCallHandler;
}
}
| packages/packages/in_app_purchase/in_app_purchase_android/android/src/main/java/io/flutter/plugins/inapppurchase/InAppPurchasePlugin.java/0 | {
"file_path": "packages/packages/in_app_purchase/in_app_purchase_android/android/src/main/java/io/flutter/plugins/inapppurchase/InAppPurchasePlugin.java",
"repo_id": "packages",
"token_count": 1034
} | 996 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:json_annotation/json_annotation.dart';
// WARNING: Changes to `@JsonSerializable` classes need to be reflected in the
// below generated file. Run `flutter packages pub run build_runner watch` to
// rebuild and watch for further changes.
part 'one_time_purchase_offer_details_wrapper.g.dart';
/// Dart wrapper around [`com.android.billingclient.api.ProductDetails.OneTimePurchaseOfferDetails`](https://developer.android.com/reference/com/android/billingclient/api/ProductDetails.OneTimePurchaseOfferDetails).
///
/// Represents the offer details to buy a one-time purchase product.
@JsonSerializable()
@immutable
class OneTimePurchaseOfferDetailsWrapper {
/// Creates a [OneTimePurchaseOfferDetailsWrapper].
@visibleForTesting
const OneTimePurchaseOfferDetailsWrapper({
required this.formattedPrice,
required this.priceAmountMicros,
required this.priceCurrencyCode,
});
/// Factory for creating a [OneTimePurchaseOfferDetailsWrapper] from a [Map]
/// with the offer details.
factory OneTimePurchaseOfferDetailsWrapper.fromJson(
Map<String, dynamic> map) =>
_$OneTimePurchaseOfferDetailsWrapperFromJson(map);
/// Formatted price for the payment, including its currency sign.
///
/// For tax exclusive countries, the price doesn't include tax.
@JsonKey(defaultValue: '')
final String formattedPrice;
/// The price for the payment in micro-units, where 1,000,000 micro-units
/// equal one unit of the currency.
///
/// For example, if price is "€7.99", price_amount_micros is "7990000". This
/// value represents the localized, rounded price for a particular currency.
@JsonKey(defaultValue: 0)
final int priceAmountMicros;
/// The ISO 4217 currency code for price.
///
/// For example, if price is specified in British pounds sterling, currency
/// code is "GBP".
@JsonKey(defaultValue: '')
final String priceCurrencyCode;
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is OneTimePurchaseOfferDetailsWrapper &&
other.formattedPrice == formattedPrice &&
other.priceAmountMicros == priceAmountMicros &&
other.priceCurrencyCode == priceCurrencyCode;
}
@override
int get hashCode {
return Object.hash(
formattedPrice.hashCode,
priceAmountMicros.hashCode,
priceCurrencyCode.hashCode,
);
}
}
| packages/packages/in_app_purchase/in_app_purchase_android/lib/src/billing_client_wrappers/one_time_purchase_offer_details_wrapper.dart/0 | {
"file_path": "packages/packages/in_app_purchase/in_app_purchase_android/lib/src/billing_client_wrappers/one_time_purchase_offer_details_wrapper.dart",
"repo_id": "packages",
"token_count": 804
} | 997 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:in_app_purchase_platform_interface/in_app_purchase_platform_interface.dart';
import '../../billing_client_wrappers.dart';
/// The class represents the information of a product as registered in at
/// Google Play store front.
class GooglePlayProductDetails extends ProductDetails {
/// Creates a new Google Play specific product details object with the
/// provided details.
GooglePlayProductDetails._({
required super.id,
required super.title,
required super.description,
required super.price,
required super.rawPrice,
required super.currencyCode,
required this.productDetails,
required super.currencySymbol,
this.subscriptionIndex,
});
/// Generates a [GooglePlayProductDetails] object based on an Android
/// [ProductDetailsWrapper] object for an in-app product.
factory GooglePlayProductDetails._fromOneTimePurchaseProductDetails(
ProductDetailsWrapper productDetails,
) {
assert(productDetails.productType == ProductType.inapp);
assert(productDetails.oneTimePurchaseOfferDetails != null);
final OneTimePurchaseOfferDetailsWrapper oneTimePurchaseOfferDetails =
productDetails.oneTimePurchaseOfferDetails!;
final String formattedPrice = oneTimePurchaseOfferDetails.formattedPrice;
final double rawPrice =
oneTimePurchaseOfferDetails.priceAmountMicros / 1000000.0;
final String currencyCode = oneTimePurchaseOfferDetails.priceCurrencyCode;
final String? currencySymbol = _extractCurrencySymbol(formattedPrice);
return GooglePlayProductDetails._(
id: productDetails.productId,
title: productDetails.title,
description: productDetails.description,
price: formattedPrice,
rawPrice: rawPrice,
currencyCode: currencyCode,
currencySymbol: currencySymbol ?? currencyCode,
productDetails: productDetails,
);
}
/// Generates a [GooglePlayProductDetails] object based on an Android
/// [ProductDetailsWrapper] object for a subscription product.
///
/// Subscriptions can consist of multiple base plans, and base plans in turn
/// can consist of multiple offers. [subscriptionIndex] points to the index of
/// [productDetails.subscriptionOfferDetails] for which the
/// [GooglePlayProductDetails] is constructed.
factory GooglePlayProductDetails._fromSubscription(
ProductDetailsWrapper productDetails,
int subscriptionIndex,
) {
assert(productDetails.productType == ProductType.subs);
assert(productDetails.subscriptionOfferDetails != null);
assert(subscriptionIndex < productDetails.subscriptionOfferDetails!.length);
final SubscriptionOfferDetailsWrapper subscriptionOfferDetails =
productDetails.subscriptionOfferDetails![subscriptionIndex];
final PricingPhaseWrapper firstPricingPhase =
subscriptionOfferDetails.pricingPhases.first;
final String formattedPrice = firstPricingPhase.formattedPrice;
final double rawPrice = firstPricingPhase.priceAmountMicros / 1000000.0;
final String currencyCode = firstPricingPhase.priceCurrencyCode;
final String? currencySymbol = _extractCurrencySymbol(formattedPrice);
return GooglePlayProductDetails._(
id: productDetails.productId,
title: productDetails.title,
description: productDetails.description,
price: formattedPrice,
rawPrice: rawPrice,
currencyCode: currencyCode,
currencySymbol: currencySymbol ?? currencyCode,
productDetails: productDetails,
subscriptionIndex: subscriptionIndex,
);
}
/// Generates a list of [GooglePlayProductDetails] based on an Android
/// [ProductDetailsWrapper] object.
///
/// If [productDetails] is of type [ProductType.inapp], a single
/// [GooglePlayProductDetails] will be constructed.
/// If [productDetails] is of type [ProductType.subs], a list is returned
/// where every element corresponds to a base plan or its offer in
/// [productDetails.subscriptionOfferDetails].
static List<GooglePlayProductDetails> fromProductDetails(
ProductDetailsWrapper productDetails,
) {
if (productDetails.productType == ProductType.inapp) {
return <GooglePlayProductDetails>[
GooglePlayProductDetails._fromOneTimePurchaseProductDetails(
productDetails),
];
} else {
final List<GooglePlayProductDetails> productDetailList =
<GooglePlayProductDetails>[];
for (int subscriptionIndex = 0;
subscriptionIndex < productDetails.subscriptionOfferDetails!.length;
subscriptionIndex++) {
productDetailList.add(GooglePlayProductDetails._fromSubscription(
productDetails,
subscriptionIndex,
));
}
return productDetailList;
}
}
/// Extracts the currency symbol from [formattedPrice].
///
/// Note that a currency symbol might consist of more than a single character.
///
/// Just in case, we assume currency symbols can appear at the start or the
/// end of [formattedPrice].
///
/// The regex captures the characters from the start/end of the [String]
/// until the first/last digit or space.
static String? _extractCurrencySymbol(String formattedPrice) {
return RegExp(r'^[^\d ]*|[^\d ]*$').firstMatch(formattedPrice)?.group(0);
}
/// Points back to the [ProductDetailsWrapper] object that was used to
/// generate this [GooglePlayProductDetails] object.
final ProductDetailsWrapper productDetails;
/// The index pointing to the [SubscriptionOfferDetailsWrapper] this
/// [GooglePlayProductDetails] object was contructed for, or `null` if it was
/// not a subscription.
///
/// The original subscription can be accessed using this index:
///
/// ```dart
/// SubscriptionOfferDetailWrapper subscription = productDetail
/// .subscriptionOfferDetails[subscriptionIndex];
/// ```
final int? subscriptionIndex;
/// The offerToken of the subscription this [GooglePlayProductDetails]
/// object was contructed for, or `null` if it was not a subscription.
String? get offerToken => subscriptionIndex != null &&
productDetails.subscriptionOfferDetails != null
? productDetails
.subscriptionOfferDetails![subscriptionIndex!].offerIdToken
: null;
}
| packages/packages/in_app_purchase/in_app_purchase_android/lib/src/types/google_play_product_details.dart/0 | {
"file_path": "packages/packages/in_app_purchase/in_app_purchase_android/lib/src/types/google_play_product_details.dart",
"repo_id": "packages",
"token_count": 1919
} | 998 |
#include "../../Flutter/Flutter-Release.xcconfig"
| packages/packages/in_app_purchase/in_app_purchase_storekit/example/macos/Runner/Configs/Release.xcconfig/0 | {
"file_path": "packages/packages/in_app_purchase/in_app_purchase_storekit/example/macos/Runner/Configs/Release.xcconfig",
"repo_id": "packages",
"token_count": 19
} | 999 |
#
# To learn more about a Podspec see http://guides.cocoapods.org/syntax/podspec.html.
# Run `pod lib lint ios_platform_images.podspec' to validate before publishing.
#
Pod::Spec.new do |s|
s.name = 'ios_platform_images'
s.version = '0.0.1'
s.summary = 'Flutter iOS Platform Images'
s.description = <<-DESC
A Flutter plugin to share images between Flutter and iOS.
Downloaded by pub (not CocoaPods).
DESC
s.homepage = 'https://github.com/flutter/packages'
s.license = { :type => 'BSD', :file => '../LICENSE' }
s.author = { 'Flutter Dev Team' => '[email protected]' }
s.source = { :http => 'https://github.com/flutter/packages/tree/main/packages/ios_platform_images' }
s.documentation_url = 'https://pub.dev/packages/ios_platform_images'
s.source_files = 'Classes/**/*.swift'
s.dependency 'Flutter'
s.platform = :ios, '12.0'
s.xcconfig = {
'DEFINES_MODULE' => 'YES',
'LIBRARY_SEARCH_PATHS' => '$(TOOLCHAIN_DIR)/usr/lib/swift/$(PLATFORM_NAME)/ $(SDKROOT)/usr/lib/swift',
'LD_RUNPATH_SEARCH_PATHS' => '/usr/lib/swift',
}
s.swift_version = '5.0'
s.resource_bundles = {'ios_platform_images_privacy' => ['Resources/PrivacyInfo.xcprivacy']}
end
| packages/packages/ios_platform_images/ios/ios_platform_images.podspec/0 | {
"file_path": "packages/packages/ios_platform_images/ios/ios_platform_images.podspec",
"repo_id": "packages",
"token_count": 571
} | 1,000 |
<resources>
<!-- Fingerprint dialog theme. -->
<!--suppress NewApi -->
<style name="AlertDialogCustom" parent="@android:style/Theme.Material.Dialog.Alert">
<item name="android:background">#FFFFFFFF</item>
<item name="android:textStyle">bold</item>
<item name="android:textSize">14sp</item>
<item name="android:colorAccent">#FF009688</item>
</style>
</resources>
| packages/packages/local_auth/local_auth_android/android/src/main/res/values/styles.xml/0 | {
"file_path": "packages/packages/local_auth/local_auth_android/android/src/main/res/values/styles.xml",
"repo_id": "packages",
"token_count": 130
} | 1,001 |
org.gradle.jvmargs=-Xmx4G
android.useAndroidX=true
android.enableJetifier=true
| packages/packages/local_auth/local_auth_android/example/android/gradle.properties/0 | {
"file_path": "packages/packages/local_auth/local_auth_android/example/android/gradle.properties",
"repo_id": "packages",
"token_count": 30
} | 1,002 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:metrics_center/src/constants.dart';
import 'package:metrics_center/src/flutter.dart';
import 'common.dart';
import 'utility.dart';
void main() {
const String gitRevision = 'ca799fa8b2254d09664b78ee80c43b434788d112';
final FlutterEngineMetricPoint simplePoint = FlutterEngineMetricPoint(
'BM_ParagraphLongLayout',
287235,
gitRevision,
);
test('FlutterEngineMetricPoint works.', () {
expect(simplePoint.value, equals(287235));
expect(simplePoint.tags[kGithubRepoKey], kFlutterEngineRepo);
expect(simplePoint.tags[kGitRevisionKey], gitRevision);
expect(simplePoint.tags[kNameKey], 'BM_ParagraphLongLayout');
final FlutterEngineMetricPoint detailedPoint = FlutterEngineMetricPoint(
'BM_ParagraphLongLayout',
287224,
'ca799fa8b2254d09664b78ee80c43b434788d112',
moreTags: const <String, String>{
'executable': 'txt_benchmarks',
'sub_result': 'CPU',
kUnitKey: 'ns',
},
);
expect(detailedPoint.value, equals(287224));
expect(detailedPoint.tags['executable'], equals('txt_benchmarks'));
expect(detailedPoint.tags['sub_result'], equals('CPU'));
expect(detailedPoint.tags[kUnitKey], equals('ns'));
});
final Map<String, dynamic>? credentialsJson = getTestGcpCredentialsJson();
test('FlutterDestination integration test with update.', () async {
final FlutterDestination dst =
await FlutterDestination.makeFromCredentialsJson(credentialsJson!,
isTesting: true);
await dst.update(<FlutterEngineMetricPoint>[simplePoint],
DateTime.fromMillisecondsSinceEpoch(123), 'test');
}, skip: credentialsJson == null);
}
| packages/packages/metrics_center/test/flutter_test.dart/0 | {
"file_path": "packages/packages/metrics_center/test/flutter_test.dart",
"repo_id": "packages",
"token_count": 680
} | 1,003 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io';
/// The IPv4 mDNS Address.
final InternetAddress mDnsAddressIPv4 = InternetAddress('224.0.0.251');
/// The IPv6 mDNS Address.
final InternetAddress mDnsAddressIPv6 = InternetAddress('FF02::FB');
/// The mDNS port.
const int mDnsPort = 5353;
/// Enumeration of supported resource record class types.
abstract class ResourceRecordClass {
// This class is intended to be used as a namespace, and should not be
// extended directly.
ResourceRecordClass._();
/// Internet address class ("IN").
static const int internet = 1;
}
/// Enumeration of DNS question types.
abstract class QuestionType {
// This class is intended to be used as a namespace, and should not be
// extended directly.
QuestionType._();
/// "QU" Question.
static const int unicast = 0x8000;
/// "QM" Question.
static const int multicast = 0x0000;
}
| packages/packages/multicast_dns/lib/src/constants.dart/0 | {
"file_path": "packages/packages/multicast_dns/lib/src/constants.dart",
"repo_id": "packages",
"token_count": 298
} | 1,004 |
#include "../../Flutter/Flutter-Debug.xcconfig"
#include "Warnings.xcconfig"
| packages/packages/palette_generator/example/macos/Runner/Configs/Debug.xcconfig/0 | {
"file_path": "packages/packages/palette_generator/example/macos/Runner/Configs/Debug.xcconfig",
"repo_id": "packages",
"token_count": 32
} | 1,005 |
#include "../../Flutter/Flutter-Debug.xcconfig"
#include "Warnings.xcconfig"
| packages/packages/path_provider/path_provider/example/macos/Runner/Configs/Debug.xcconfig/0 | {
"file_path": "packages/packages/path_provider/path_provider/example/macos/Runner/Configs/Debug.xcconfig",
"repo_id": "packages",
"token_count": 32
} | 1,006 |
## NEXT
* Updates minimum supported SDK version to Flutter 3.13/Dart 3.1.
* Updates compileSdk version to 34.
## 2.2.2
* Updates minimum supported SDK version to Flutter 3.10/Dart 3.0.
* Updates annotations lib to 1.7.1.
## 2.2.1
* Updates annotations lib to 1.7.0.
## 2.2.0
* Adds implementation of `getDownloadsDirectory()`.
## 2.1.1
* Adds pub topics to package metadata.
* Updates minimum supported SDK version to Flutter 3.7/Dart 2.19.
## 2.1.0
* Adds getApplicationCachePath() for storing app-specific cache files.
* Updates minimum supported SDK version to Flutter 3.3/Dart 2.18.
## 2.0.27
* Fixes compatibility with AGP versions older than 4.2.
## 2.0.26
* Adds a namespace for compatibility with AGP 8.0.
## 2.0.25
* Fixes Java warnings.
## 2.0.24
* Clarifies explanation of endorsement in README.
* Aligns Dart and Flutter SDK constraints.
* Updates compileSdkVersion to 33.
## 2.0.23
* Updates links for the merge of flutter/plugins into flutter/packages.
* Updates minimum Flutter version to 3.0.
## 2.0.22
* Removes unused Guava dependency.
## 2.0.21
* Updates code for `no_leading_underscores_for_local_identifiers` lint.
* Updates minimum Flutter version to 2.10.
* Upgrades `androidx.annotation` version to 1.5.0.
* Upgrades Android Gradle plugin version to 7.3.1.
## 2.0.20
* Reverts changes in versions 2.0.18 and 2.0.19.
## 2.0.19
* Bumps kotlin to 1.7.10
## 2.0.18
* Bumps `androidx.annotation:annotation` version to 1.4.0.
* Bumps gradle version to 7.2.2.
## 2.0.17
* Lower minimim version back to 2.8.1.
## 2.0.16
* Fixes bug with `getExternalStoragePaths(null)`.
## 2.0.15
* Switches the medium from MethodChannels to Pigeon.
## 2.0.14
* Fixes library_private_types_in_public_api, sort_child_properties_last and use_key_in_widget_constructors
lint warnings.
## 2.0.13
* Fixes typing build warning.
## 2.0.12
* Returns to using a different platform channel name, undoing the revert in
2.0.11, but updates the minimum Flutter version to 2.8 to avoid the issue
that caused the revert.
## 2.0.11
* Temporarily reverts the platform channel name change from 2.0.10 in order to
restore compatibility with Flutter versions earlier than 2.8.
## 2.0.10
* Switches to a package-internal implementation of the platform interface.
## 2.0.9
* Updates Android compileSdkVersion to 31.
## 2.0.8
* Updates example app Android compileSdkVersion to 31.
* Fixes typing build warning.
## 2.0.7
* Fixes link in README.
## 2.0.6
* Split from `path_provider` as a federated implementation.
| packages/packages/path_provider/path_provider_android/CHANGELOG.md/0 | {
"file_path": "packages/packages/path_provider/path_provider_android/CHANGELOG.md",
"repo_id": "packages",
"token_count": 884
} | 1,007 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package dev.flutter.pigeon_example_app
import ExampleHostApi
import FlutterError
import MessageData
import MessageFlutterApi
import androidx.annotation.NonNull
import io.flutter.embedding.android.FlutterActivity
import io.flutter.embedding.engine.FlutterEngine
import io.flutter.embedding.engine.plugins.FlutterPlugin
// #docregion kotlin-class
private class PigeonApiImplementation : ExampleHostApi {
override fun getHostLanguage(): String {
return "Kotlin"
}
override fun add(a: Long, b: Long): Long {
if (a < 0L || b < 0L) {
throw FlutterError("code", "message", "details")
}
return a + b
}
override fun sendMessage(message: MessageData, callback: (Result<Boolean>) -> Unit) {
if (message.code == Code.ONE) {
callback(Result.failure(FlutterError("code", "message", "details")))
return
}
callback(Result.success(true))
}
}
// #enddocregion kotlin-class
// #docregion kotlin-class-flutter
private class PigeonFlutterApi {
var flutterApi: MessageFlutterApi? = null
constructor(binding: FlutterPlugin.FlutterPluginBinding) {
flutterApi = MessageFlutterApi(binding.getBinaryMessenger())
}
fun callFlutterMethod(aString: String, callback: (Result<String>) -> Unit) {
flutterApi!!.flutterMethod(aString) { echo -> callback(Result.success(echo)) }
}
}
// #enddocregion kotlin-class-flutter
class MainActivity : FlutterActivity() {
override fun configureFlutterEngine(@NonNull flutterEngine: FlutterEngine) {
super.configureFlutterEngine(flutterEngine)
val api = PigeonApiImplementation()
ExampleHostApi.setUp(flutterEngine.dartExecutor.binaryMessenger, api)
}
}
| packages/packages/pigeon/example/app/android/app/src/main/kotlin/dev/flutter/pigeon_example_app/MainActivity.kt/0 | {
"file_path": "packages/packages/pigeon/example/app/android/app/src/main/kotlin/dev/flutter/pigeon_example_app/MainActivity.kt",
"repo_id": "packages",
"token_count": 610
} | 1,008 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_test/flutter_test.dart';
import 'package:integration_test/integration_test.dart';
import 'package:pigeon_example_app/main.dart';
void main() {
IntegrationTestWidgetsFlutterBinding.ensureInitialized();
testWidgets('gets host language', (WidgetTester tester) async {
await tester.pumpWidget(const MyApp());
await tester.pumpAndSettle();
expect(find.textContaining('Hello from'), findsOneWidget);
});
}
| packages/packages/pigeon/example/app/integration_test/example_app_test.dart/0 | {
"file_path": "packages/packages/pigeon/example/app/integration_test/example_app_test.dart",
"repo_id": "packages",
"token_count": 192
} | 1,009 |
#include "../../Flutter/Flutter-Release.xcconfig"
#include "Warnings.xcconfig"
| packages/packages/pigeon/example/app/macos/Runner/Configs/Release.xcconfig/0 | {
"file_path": "packages/packages/pigeon/example/app/macos/Runner/Configs/Release.xcconfig",
"repo_id": "packages",
"token_count": 32
} | 1,010 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:collection/collection.dart' show ListEquality;
import 'package:meta/meta.dart';
import 'pigeon_lib.dart';
typedef _ListEquals = bool Function(List<Object?>, List<Object?>);
final _ListEquals _listEquals = const ListEquality<dynamic>().equals;
/// Enum that represents where an [Api] is located, on the host or Flutter.
enum ApiLocation {
/// The API is for calling functions defined on the host.
host,
/// The API is for calling functions defined in Flutter.
flutter,
}
/// Superclass for all AST nodes.
class Node {}
/// Represents a method on an [Api].
class Method extends Node {
/// Parametric constructor for [Method].
Method({
required this.name,
required this.returnType,
required this.parameters,
required this.location,
this.isRequired = true,
this.isAsynchronous = false,
this.isStatic = false,
this.offset,
this.objcSelector = '',
this.swiftFunction = '',
this.taskQueueType = TaskQueueType.serial,
this.documentationComments = const <String>[],
});
/// The name of the method.
String name;
/// The data-type of the return value.
TypeDeclaration returnType;
/// The parameters passed into the [Method].
List<Parameter> parameters;
/// Whether the receiver of this method is expected to return synchronously or not.
bool isAsynchronous;
/// The offset in the source file where the field appears.
int? offset;
/// An override for the generated objc selector (ex. "divideNumber:by:").
String objcSelector;
/// An override for the generated swift function signature (ex. "divideNumber(_:by:)").
String swiftFunction;
/// Specifies how handlers are dispatched with respect to threading.
TaskQueueType taskQueueType;
/// List of documentation comments, separated by line.
///
/// Lines should not include the comment marker itself, but should include any
/// leading whitespace, so that any indentation in the original comment is preserved.
/// For example: [" List of documentation comments, separated by line.", ...]
List<String> documentationComments;
/// Where the implementation of this method is located, host or Flutter.
ApiLocation location;
/// Whether this method is required to be implemented.
///
/// This flag is typically used to determine whether a callback method for
/// a `ProxyApi` is nullable or not.
bool isRequired;
/// Whether this is a static method of a ProxyApi.
bool isStatic;
@override
String toString() {
final String objcSelectorStr =
objcSelector.isEmpty ? '' : ' objcSelector:$objcSelector';
final String swiftFunctionStr =
swiftFunction.isEmpty ? '' : ' swiftFunction:$swiftFunction';
return '(Method name:$name returnType:$returnType parameters:$parameters isAsynchronous:$isAsynchronous$objcSelectorStr$swiftFunctionStr documentationComments:$documentationComments)';
}
}
/// Represents a collection of [Method]s that are implemented on the platform
/// side.
class AstHostApi extends Api {
/// Parametric constructor for [AstHostApi].
AstHostApi({
required super.name,
required super.methods,
super.documentationComments = const <String>[],
this.dartHostTestHandler,
});
/// The name of the Dart test interface to generate to help with testing.
String? dartHostTestHandler;
@override
String toString() {
return '(HostApi name:$name methods:$methods documentationComments:$documentationComments dartHostTestHandler:$dartHostTestHandler)';
}
}
/// Represents a collection of [Method]s that are hosted on the Flutter side.
class AstFlutterApi extends Api {
/// Parametric constructor for [AstFlutterApi].
AstFlutterApi({
required super.name,
required super.methods,
super.documentationComments = const <String>[],
});
@override
String toString() {
return '(FlutterApi name:$name methods:$methods documentationComments:$documentationComments)';
}
}
/// Represents an API that wraps a native class.
class AstProxyApi extends Api {
/// Parametric constructor for [AstProxyApi].
AstProxyApi({
required super.name,
required super.methods,
super.documentationComments = const <String>[],
required this.constructors,
required this.fields,
this.superClass,
this.interfaces = const <TypeDeclaration>{},
});
/// List of constructors inside the API.
final List<Constructor> constructors;
/// List of fields inside the API.
List<ApiField> fields;
/// Name of the class this class considers the super class.
TypeDeclaration? superClass;
/// Name of the classes this class considers to be implemented.
Set<TypeDeclaration> interfaces;
/// Methods implemented in the host platform language.
Iterable<Method> get hostMethods => methods.where(
(Method method) => method.location == ApiLocation.host,
);
/// Methods implemented in Flutter.
Iterable<Method> get flutterMethods => methods.where(
(Method method) => method.location == ApiLocation.flutter,
);
/// All fields that are attached.
///
/// See [attached].
Iterable<ApiField> get attachedFields => fields.where(
(ApiField field) => field.isAttached,
);
/// All fields that are not attached.
///
/// See [attached].
Iterable<ApiField> get unattachedFields => fields.where(
(ApiField field) => !field.isAttached,
);
/// A list of AstProxyApis where each `extends` the API that follows it.
///
/// Returns an empty list if this api does not extend a ProxyApi.
///
/// This method assumes the super classes of each ProxyApi doesn't create a
/// loop. Throws a [ArgumentError] if a loop is found.
///
/// This method also assumes that all super classes are ProxyApis. Otherwise,
/// throws an [ArgumentError].
Iterable<AstProxyApi> allSuperClasses() {
final List<AstProxyApi> superClassChain = <AstProxyApi>[];
if (superClass != null && !superClass!.isProxyApi) {
throw ArgumentError(
'Could not find a ProxyApi for super class: ${superClass!.baseName}',
);
}
AstProxyApi? currentProxyApi = superClass?.associatedProxyApi;
while (currentProxyApi != null) {
if (superClassChain.contains(currentProxyApi)) {
throw ArgumentError(
'Loop found when processing super classes for a ProxyApi: '
'$name, ${superClassChain.map((AstProxyApi api) => api.name)}',
);
}
superClassChain.add(currentProxyApi);
if (currentProxyApi.superClass != null &&
!currentProxyApi.superClass!.isProxyApi) {
throw ArgumentError(
'Could not find a ProxyApi for super class: '
'${currentProxyApi.superClass!.baseName}',
);
}
currentProxyApi = currentProxyApi.superClass?.associatedProxyApi;
}
return superClassChain;
}
/// All ProxyApis this API `implements` and all the interfaces those APIs
/// `implements`.
Iterable<AstProxyApi> apisOfInterfaces() => _recursiveFindAllInterfaceApis();
/// All methods inherited from interfaces and the interfaces of interfaces.
Iterable<Method> flutterMethodsFromInterfaces() sync* {
for (final AstProxyApi proxyApi in apisOfInterfaces()) {
yield* proxyApi.methods;
}
}
/// A list of Flutter methods inherited from the ProxyApi that this ProxyApi
/// `extends`.
///
/// This also recursively checks the ProxyApi that the super class `extends`
/// and so on.
///
/// This also includes methods that super classes inherited from interfaces
/// with `implements`.
Iterable<Method> flutterMethodsFromSuperClasses() sync* {
for (final AstProxyApi proxyApi in allSuperClasses().toList().reversed) {
yield* proxyApi.flutterMethods;
}
if (superClass != null) {
final Set<AstProxyApi> interfaceApisFromSuperClasses =
superClass!.associatedProxyApi!._recursiveFindAllInterfaceApis();
for (final AstProxyApi proxyApi in interfaceApisFromSuperClasses) {
yield* proxyApi.methods;
}
}
}
/// Whether the api has a method that callbacks to Dart to add a new instance
/// to the InstanceManager.
///
/// This is possible as long as no callback methods are required to
/// instantiate the class.
bool hasCallbackConstructor() {
return flutterMethods
.followedBy(flutterMethodsFromSuperClasses())
.followedBy(flutterMethodsFromInterfaces())
.every((Method method) => !method.isRequired);
}
// Recursively search for all the interfaces apis from a list of names of
// interfaces.
//
// This method assumes that all interfaces are ProxyApis and an api doesn't
// contains itself as an interface. Otherwise, throws an [ArgumentError].
Set<AstProxyApi> _recursiveFindAllInterfaceApis([
Set<AstProxyApi> seenApis = const <AstProxyApi>{},
]) {
final Set<AstProxyApi> allInterfaces = <AstProxyApi>{};
allInterfaces.addAll(
interfaces.map(
(TypeDeclaration type) {
if (!type.isProxyApi) {
throw ArgumentError(
'Could not find a valid ProxyApi for an interface: $type',
);
} else if (seenApis.contains(type.associatedProxyApi)) {
throw ArgumentError(
'A ProxyApi cannot be a super class of itself: ${type.baseName}',
);
}
return type.associatedProxyApi!;
},
),
);
// Adds the current api since it would be invalid for it to be an interface
// of itself.
final Set<AstProxyApi> newSeenApis = <AstProxyApi>{...seenApis, this};
for (final AstProxyApi interfaceApi in <AstProxyApi>{...allInterfaces}) {
allInterfaces.addAll(
interfaceApi._recursiveFindAllInterfaceApis(newSeenApis),
);
}
return allInterfaces;
}
@override
String toString() {
return '(ProxyApi name:$name methods:$methods field:$fields '
'documentationComments:$documentationComments '
'superClassName:$superClass interfacesNames:$interfaces)';
}
}
/// Represents a constructor for an API.
class Constructor extends Method {
/// Parametric constructor for [Constructor].
Constructor({
required super.name,
required super.parameters,
super.offset,
super.swiftFunction = '',
super.documentationComments = const <String>[],
}) : super(
returnType: const TypeDeclaration.voidDeclaration(),
location: ApiLocation.host,
);
@override
String toString() {
final String swiftFunctionStr =
swiftFunction.isEmpty ? '' : ' swiftFunction:$swiftFunction';
return '(Constructor name:$name parameters:$parameters $swiftFunctionStr documentationComments:$documentationComments)';
}
}
/// Represents a field of an API.
class ApiField extends NamedType {
/// Constructor for [ApiField].
ApiField({
required super.name,
required super.type,
super.offset,
super.documentationComments,
this.isAttached = false,
this.isStatic = false,
}) : assert(!isStatic || isAttached);
/// Whether this is an attached field for a [AstProxyApi].
///
/// See [attached].
final bool isAttached;
/// Whether this is a static field of a [AstProxyApi].
///
/// A static field must also be attached. See [attached].
final bool isStatic;
/// Returns a copy of [Parameter] instance with new attached [TypeDeclaration].
@override
ApiField copyWithType(TypeDeclaration type) {
return ApiField(
name: name,
type: type,
offset: offset,
documentationComments: documentationComments,
isAttached: isAttached,
isStatic: isStatic,
);
}
@override
String toString() {
return '(Field name:$name type:$type isAttached:$isAttached '
'isStatic:$isStatic documentationComments:$documentationComments)';
}
}
/// Represents a collection of [Method]s.
sealed class Api extends Node {
/// Parametric constructor for [Api].
Api({
required this.name,
required this.methods,
this.documentationComments = const <String>[],
});
/// The name of the API.
String name;
/// List of methods inside the API.
List<Method> methods;
/// List of documentation comments, separated by line.
///
/// Lines should not include the comment marker itself, but should include any
/// leading whitespace, so that any indentation in the original comment is preserved.
/// For example: [" List of documentation comments, separated by line.", ...]
List<String> documentationComments;
@override
String toString() {
return '(Api name:$name methods:$methods documentationComments:$documentationComments)';
}
}
/// A specific instance of a type.
@immutable
class TypeDeclaration {
/// Constructor for [TypeDeclaration].
const TypeDeclaration({
required this.baseName,
required this.isNullable,
this.associatedEnum,
this.associatedClass,
this.associatedProxyApi,
this.typeArguments = const <TypeDeclaration>[],
});
/// Void constructor.
const TypeDeclaration.voidDeclaration()
: baseName = 'void',
isNullable = false,
associatedEnum = null,
associatedClass = null,
associatedProxyApi = null,
typeArguments = const <TypeDeclaration>[];
/// The base name of the [TypeDeclaration] (ex 'Foo' to 'Foo<Bar>?').
final String baseName;
/// Whether the declaration represents 'void'.
bool get isVoid => baseName == 'void';
/// Whether the type arguments to the entity (ex 'Bar' to 'Foo<Bar>?').
final List<TypeDeclaration> typeArguments;
/// Whether the type is nullable.
final bool isNullable;
/// Whether the [TypeDeclaration] has an [associatedEnum].
bool get isEnum => associatedEnum != null;
/// Associated [Enum], if any.
final Enum? associatedEnum;
/// Whether the [TypeDeclaration] has an [associatedClass].
bool get isClass => associatedClass != null;
/// Associated [Class], if any.
final Class? associatedClass;
/// Whether the [TypeDeclaration] has an [associatedProxyApi].
bool get isProxyApi => associatedProxyApi != null;
/// Associated [AstProxyApi], if any.
final AstProxyApi? associatedProxyApi;
@override
int get hashCode {
// This has to be implemented because TypeDeclaration is used as a Key to a
// Map in generator_tools.dart.
int hash = 17;
hash = hash * 37 + baseName.hashCode;
hash = hash * 37 + isNullable.hashCode;
for (final TypeDeclaration typeArgument in typeArguments) {
hash = hash * 37 + typeArgument.hashCode;
}
return hash;
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
} else {
return other is TypeDeclaration &&
baseName == other.baseName &&
isNullable == other.isNullable &&
_listEquals(typeArguments, other.typeArguments) &&
isEnum == other.isEnum &&
isClass == other.isClass &&
associatedClass == other.associatedClass &&
associatedEnum == other.associatedEnum;
}
}
/// Returns duplicated `TypeDeclaration` with attached `associatedEnum` value.
TypeDeclaration copyWithEnum(Enum enumDefinition) {
return TypeDeclaration(
baseName: baseName,
isNullable: isNullable,
associatedEnum: enumDefinition,
typeArguments: typeArguments,
);
}
/// Returns duplicated `TypeDeclaration` with attached `associatedClass` value.
TypeDeclaration copyWithClass(Class classDefinition) {
return TypeDeclaration(
baseName: baseName,
isNullable: isNullable,
associatedClass: classDefinition,
typeArguments: typeArguments,
);
}
/// Returns duplicated `TypeDeclaration` with attached `associatedProxyApi` value.
TypeDeclaration copyWithProxyApi(AstProxyApi proxyApiDefinition) {
return TypeDeclaration(
baseName: baseName,
isNullable: isNullable,
associatedProxyApi: proxyApiDefinition,
typeArguments: typeArguments,
);
}
@override
String toString() {
final String typeArgumentsStr =
typeArguments.isEmpty ? '' : 'typeArguments:$typeArguments';
return '(TypeDeclaration baseName:$baseName isNullable:$isNullable$typeArgumentsStr isEnum:$isEnum isClass:$isClass isProxyApi:$isProxyApi)';
}
}
/// Represents a named entity that has a type.
@immutable
class NamedType extends Node {
/// Parametric constructor for [NamedType].
NamedType({
required this.name,
required this.type,
this.offset,
this.defaultValue,
this.documentationComments = const <String>[],
});
/// The name of the entity.
final String name;
/// The type.
final TypeDeclaration type;
/// The offset in the source file where the [NamedType] appears.
final int? offset;
/// Stringified version of the default value of types that have default values.
final String? defaultValue;
/// List of documentation comments, separated by line.
///
/// Lines should not include the comment marker itself, but should include any
/// leading whitespace, so that any indentation in the original comment is preserved.
/// For example: [" List of documentation comments, separated by line.", ...]
final List<String> documentationComments;
/// Returns a copy of [NamedType] instance with new attached [TypeDeclaration].
@mustBeOverridden
NamedType copyWithType(TypeDeclaration type) {
return NamedType(
name: name,
type: type,
offset: offset,
defaultValue: defaultValue,
documentationComments: documentationComments,
);
}
@override
String toString() {
return '(NamedType name:$name type:$type defaultValue:$defaultValue documentationComments:$documentationComments)';
}
}
/// Represents a [Method]'s parameter that has a type and a name.
@immutable
class Parameter extends NamedType {
/// Parametric constructor for [Parameter].
Parameter({
required super.name,
required super.type,
super.offset,
super.defaultValue,
bool? isNamed,
bool? isOptional,
bool? isPositional,
bool? isRequired,
super.documentationComments,
}) : isNamed = isNamed ?? false,
isOptional = isOptional ?? false,
isPositional = isPositional ?? true,
isRequired = isRequired ?? true;
/// Whether this parameter is a named parameter.
///
/// Defaults to `true`.
final bool isNamed;
/// Whether this parameter is an optional parameter.
///
/// Defaults to `false`.
final bool isOptional;
/// Whether this parameter is a positional parameter.
///
/// Defaults to `true`.
final bool isPositional;
/// Whether this parameter is a required parameter.
///
/// Defaults to `true`.
final bool isRequired;
/// Returns a copy of [Parameter] instance with new attached [TypeDeclaration].
@override
Parameter copyWithType(TypeDeclaration type) {
return Parameter(
name: name,
type: type,
offset: offset,
defaultValue: defaultValue,
isNamed: isNamed,
isOptional: isOptional,
isPositional: isPositional,
isRequired: isRequired,
documentationComments: documentationComments,
);
}
@override
String toString() {
return '(Parameter name:$name type:$type isNamed:$isNamed isOptional:$isOptional isPositional:$isPositional isRequired:$isRequired documentationComments:$documentationComments)';
}
}
/// Represents a class with fields.
class Class extends Node {
/// Parametric constructor for [Class].
Class({
required this.name,
required this.fields,
this.documentationComments = const <String>[],
});
/// The name of the class.
String name;
/// All the fields contained in the class.
List<NamedType> fields;
/// List of documentation comments, separated by line.
///
/// Lines should not include the comment marker itself, but should include any
/// leading whitespace, so that any indentation in the original comment is preserved.
/// For example: [" List of documentation comments, separated by line.", ...]
List<String> documentationComments;
@override
String toString() {
return '(Class name:$name fields:$fields documentationComments:$documentationComments)';
}
}
/// Represents a Enum.
class Enum extends Node {
/// Parametric constructor for [Enum].
Enum({
required this.name,
required this.members,
this.documentationComments = const <String>[],
});
/// The name of the enum.
String name;
/// All of the members of the enum.
List<EnumMember> members;
/// List of documentation comments, separated by line.
///
/// Lines should not include the comment marker itself, but should include any
/// leading whitespace, so that any indentation in the original comment is preserved.
/// For example: [" List of documentation comments, separated by line.", ...]
List<String> documentationComments;
@override
String toString() {
return '(Enum name:$name members:$members documentationComments:$documentationComments)';
}
}
/// Represents a Enum member.
class EnumMember extends Node {
/// Parametric constructor for [EnumMember].
EnumMember({
required this.name,
this.documentationComments = const <String>[],
});
/// The name of the enum member.
final String name;
/// List of documentation comments, separated by line.
///
/// Lines should not include the comment marker itself, but should include any
/// leading whitespace, so that any indentation in the original comment is preserved.
/// For example: [" List of documentation comments, separated by line.", ...]
final List<String> documentationComments;
@override
String toString() {
return '(EnumMember name:$name documentationComments:$documentationComments)';
}
}
/// Top-level node for the AST.
class Root extends Node {
/// Parametric constructor for [Root].
Root({
required this.classes,
required this.apis,
required this.enums,
});
/// Factory function for generating an empty root, usually used when early errors are encountered.
factory Root.makeEmpty() {
return Root(apis: <Api>[], classes: <Class>[], enums: <Enum>[]);
}
/// All the classes contained in the AST.
List<Class> classes;
/// All the API's contained in the AST.
List<Api> apis;
/// All of the enums contained in the AST.
List<Enum> enums;
@override
String toString() {
return '(Root classes:$classes apis:$apis enums:$enums)';
}
}
| packages/packages/pigeon/lib/ast.dart/0 | {
"file_path": "packages/packages/pigeon/lib/ast.dart",
"repo_id": "packages",
"token_count": 7288
} | 1,011 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.