text
stringlengths 6
13.6M
| id
stringlengths 13
176
| metadata
dict | __index_level_0__
int64 0
1.69k
|
---|---|---|---|
import 'package:flame/components.dart';
import 'package:flutter/material.dart';
import 'package:pinball/game/game.dart';
import 'package:pinball_components/pinball_components.dart';
import 'package:pinball_ui/pinball_ui.dart';
final _bodyTextPaint = TextPaint(
style: const TextStyle(
fontSize: 3,
color: PinballColors.white,
fontFamily: PinballFonts.pixeloidSans,
),
);
/// {@template initials_submission_success_display}
/// [Backbox] display for initials successfully submitted.
/// {@endtemplate}
class InitialsSubmissionSuccessDisplay extends TextComponent {
@override
Future<void> onLoad() async {
await super.onLoad();
position = Vector2(0, -10);
anchor = Anchor.center;
text = 'Success!';
textRenderer = _bodyTextPaint;
}
}
| pinball/lib/game/components/backbox/displays/initials_submission_success_display.dart/0 | {
"file_path": "pinball/lib/game/components/backbox/displays/initials_submission_success_display.dart",
"repo_id": "pinball",
"token_count": 269
} | 1,150 |
import 'package:flame/components.dart';
import 'package:flame_bloc/flame_bloc.dart';
import 'package:pinball/game/game.dart';
import 'package:pinball/select_character/select_character.dart';
import 'package:pinball_audio/pinball_audio.dart';
import 'package:pinball_components/pinball_components.dart';
import 'package:pinball_flame/pinball_flame.dart';
/// Listens to the [GameBloc] and updates the game accordingly.
class GameBlocStatusListener extends Component
with FlameBlocListenable<GameBloc, GameState>, HasGameRef {
@override
bool listenWhen(GameState? previousState, GameState newState) {
return previousState?.status != newState.status;
}
@override
void onNewState(GameState state) {
switch (state.status) {
case GameStatus.waiting:
break;
case GameStatus.playing:
readProvider<PinballAudioPlayer>().play(PinballAudio.backgroundMusic);
_resetBonuses();
gameRef
.descendants()
.whereType<Flipper>()
.forEach(_addFlipperBehaviors);
gameRef
.descendants()
.whereType<Plunger>()
.forEach(_addPlungerBehaviors);
gameRef.overlays.remove(PinballGame.playButtonOverlay);
gameRef.overlays.remove(PinballGame.replayButtonOverlay);
break;
case GameStatus.gameOver:
readProvider<PinballAudioPlayer>().play(PinballAudio.gameOverVoiceOver);
gameRef.descendants().whereType<Backbox>().first.requestInitials(
score: state.displayScore,
character: readBloc<CharacterThemeCubit, CharacterThemeState>()
.state
.characterTheme,
);
gameRef
.descendants()
.whereType<Flipper>()
.forEach(_removeFlipperBehaviors);
gameRef
.descendants()
.whereType<Plunger>()
.forEach(_removePlungerBehaviors);
break;
}
}
void _resetBonuses() {
gameRef
.descendants()
.whereType<FlameBlocProvider<GoogleWordCubit, GoogleWordState>>()
.single
.bloc
.onReset();
gameRef
.descendants()
.whereType<FlameBlocProvider<DashBumpersCubit, DashBumpersState>>()
.single
.bloc
.onReset();
gameRef
.descendants()
.whereType<FlameBlocProvider<SignpostCubit, SignpostState>>()
.single
.bloc
.onReset();
}
void _addPlungerBehaviors(Plunger plunger) => plunger
.firstChild<FlameBlocProvider<PlungerCubit, PlungerState>>()!
.addAll(
[
PlungerPullingBehavior(strength: 7),
PlungerAutoPullingBehavior(),
PlungerKeyControllingBehavior()
],
);
void _removePlungerBehaviors(Plunger plunger) {
plunger
.descendants()
.whereType<PlungerPullingBehavior>()
.forEach(plunger.remove);
plunger
.descendants()
.whereType<PlungerAutoPullingBehavior>()
.forEach(plunger.remove);
plunger
.descendants()
.whereType<PlungerKeyControllingBehavior>()
.forEach(plunger.remove);
}
void _addFlipperBehaviors(Flipper flipper) => flipper
.firstChild<FlameBlocProvider<FlipperCubit, FlipperState>>()!
.add(FlipperKeyControllingBehavior());
void _removeFlipperBehaviors(Flipper flipper) => flipper
.descendants()
.whereType<FlipperKeyControllingBehavior>()
.forEach(flipper.remove);
}
| pinball/lib/game/components/game_bloc_status_listener.dart/0 | {
"file_path": "pinball/lib/game/components/game_bloc_status_listener.dart",
"repo_id": "pinball",
"token_count": 1592
} | 1,151 |
import 'dart:async';
import 'package:flame/components.dart';
import 'package:flame/game.dart';
import 'package:flame/input.dart';
import 'package:flame_bloc/flame_bloc.dart';
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
import 'package:leaderboard_repository/leaderboard_repository.dart';
import 'package:pinball/game/behaviors/behaviors.dart';
import 'package:pinball/game/game.dart';
import 'package:pinball/l10n/l10n.dart';
import 'package:pinball/select_character/select_character.dart';
import 'package:pinball_audio/pinball_audio.dart';
import 'package:pinball_components/pinball_components.dart';
import 'package:pinball_flame/pinball_flame.dart';
import 'package:platform_helper/platform_helper.dart';
import 'package:share_repository/share_repository.dart';
class PinballGame extends PinballForge2DGame
with HasKeyboardHandlerComponents, MultiTouchTapDetector, HasTappables {
PinballGame({
required CharacterThemeCubit characterThemeBloc,
required this.leaderboardRepository,
required this.shareRepository,
required GameBloc gameBloc,
required AppLocalizations l10n,
required PinballAudioPlayer audioPlayer,
required this.platformHelper,
}) : focusNode = FocusNode(),
_gameBloc = gameBloc,
_audioPlayer = audioPlayer,
_characterThemeBloc = characterThemeBloc,
_l10n = l10n,
super(
gravity: Vector2(0, 30),
) {
images.prefix = '';
}
/// Identifier of the play button overlay.
static const playButtonOverlay = 'play_button';
/// Identifier of the replay button overlay.
static const replayButtonOverlay = 'replay_button';
/// Identifier of the mobile controls overlay.
static const mobileControlsOverlay = 'mobile_controls';
@override
Color backgroundColor() => Colors.transparent;
final FocusNode focusNode;
final CharacterThemeCubit _characterThemeBloc;
final PinballAudioPlayer _audioPlayer;
final LeaderboardRepository leaderboardRepository;
final ShareRepository shareRepository;
final AppLocalizations _l10n;
final PlatformHelper platformHelper;
final GameBloc _gameBloc;
List<LeaderboardEntryData>? _entries;
Future<void> preFetchLeaderboard() async {
try {
_entries = await leaderboardRepository.fetchTop10Leaderboard();
} catch (_) {
// An initial null leaderboard means that we couldn't fetch
// the entries for the [Backbox] and it will show the relevant display.
_entries = null;
}
}
@override
Future<void> onLoad() async {
await add(
FlameMultiBlocProvider(
providers: [
FlameBlocProvider<GameBloc, GameState>.value(
value: _gameBloc,
),
FlameBlocProvider<CharacterThemeCubit, CharacterThemeState>.value(
value: _characterThemeBloc,
),
],
children: [
MultiFlameProvider(
providers: [
FlameProvider<PinballAudioPlayer>.value(_audioPlayer),
FlameProvider<LeaderboardRepository>.value(leaderboardRepository),
FlameProvider<ShareRepository>.value(shareRepository),
FlameProvider<AppLocalizations>.value(_l10n),
FlameProvider<PlatformHelper>.value(platformHelper),
],
children: [
BonusNoiseBehavior(),
GameBlocStatusListener(),
BallSpawningBehavior(),
CharacterSelectionBehavior(),
CameraFocusingBehavior(),
CanvasComponent(
onSpritePainted: (paint) {
if (paint.filterQuality != FilterQuality.medium) {
paint.filterQuality = FilterQuality.medium;
}
},
children: [
ZCanvasComponent(
children: [
if (!platformHelper.isMobile) ArcadeBackground(),
BoardBackgroundSpriteComponent(),
Boundaries(),
Backbox(
leaderboardRepository: leaderboardRepository,
shareRepository: shareRepository,
entries: _entries,
),
GoogleGallery(),
Multipliers(),
Multiballs(),
SkillShot(
children: [
ScoringContactBehavior(points: Points.oneMillion),
RolloverNoiseBehavior(),
],
),
AndroidAcres(),
DinoDesert(),
FlutterForest(),
SparkyScorch(),
Drain(),
BottomGroup(),
Launcher(),
],
),
],
),
],
),
],
),
);
await super.onLoad();
}
final focusedBoardSide = <int, BoardSide>{};
@override
void onTapDown(int pointerId, TapDownInfo info) {
if (info.raw.kind == PointerDeviceKind.touch &&
_gameBloc.state.status.isPlaying) {
final rocket = descendants().whereType<RocketSpriteComponent>().first;
final bounds = rocket.topLeftPosition & rocket.size;
final tappedRocket = bounds.contains(info.eventPosition.game.toOffset());
if (tappedRocket) {
descendants()
.whereType<FlameBlocProvider<PlungerCubit, PlungerState>>()
.first
.bloc
.autoPulled();
} else {
final tappedLeftSide = info.eventPosition.widget.x < canvasSize.x / 2;
focusedBoardSide[pointerId] =
tappedLeftSide ? BoardSide.left : BoardSide.right;
final flippers = descendants()
.whereType<Flipper>()
.where((flipper) => flipper.side == focusedBoardSide[pointerId]);
for (final flipper in flippers) {
flipper
.descendants()
.whereType<FlameBlocProvider<FlipperCubit, FlipperState>>()
.forEach((provider) => provider.bloc.moveUp());
}
}
}
super.onTapDown(pointerId, info);
}
@override
void onTapUp(int pointerId, TapUpInfo info) {
_moveFlippersDown(pointerId);
super.onTapUp(pointerId, info);
}
@override
void onTapCancel(int pointerId) {
_moveFlippersDown(pointerId);
super.onTapCancel(pointerId);
}
void _moveFlippersDown(int pointerId) {
if (focusedBoardSide[pointerId] != null) {
final flippers = descendants()
.whereType<Flipper>()
.where((flipper) => flipper.side == focusedBoardSide[pointerId]);
for (final flipper in flippers) {
flipper
.descendants()
.whereType<FlameBlocProvider<FlipperCubit, FlipperState>>()
.forEach((provider) => provider.bloc.moveDown());
}
}
}
}
class DebugPinballGame extends PinballGame with FPSCounter, PanDetector {
DebugPinballGame({
required CharacterThemeCubit characterThemeBloc,
required LeaderboardRepository leaderboardRepository,
required ShareRepository shareRepository,
required AppLocalizations l10n,
required PinballAudioPlayer audioPlayer,
required PlatformHelper platformHelper,
required GameBloc gameBloc,
}) : super(
characterThemeBloc: characterThemeBloc,
audioPlayer: audioPlayer,
leaderboardRepository: leaderboardRepository,
shareRepository: shareRepository,
l10n: l10n,
platformHelper: platformHelper,
gameBloc: gameBloc,
);
Vector2? lineStart;
Vector2? lineEnd;
@override
Future<void> onLoad() async {
await super.onLoad();
await addAll([PreviewLine(), _DebugInformation()]);
}
@override
void onTapUp(int pointerId, TapUpInfo info) {
super.onTapUp(pointerId, info);
if (info.raw.kind == PointerDeviceKind.mouse) {
final canvas = descendants().whereType<ZCanvasComponent>().single;
final ball = Ball()..initialPosition = info.eventPosition.game;
canvas.add(ball);
}
}
@override
void onPanStart(DragStartInfo info) => lineStart = info.eventPosition.game;
@override
void onPanUpdate(DragUpdateInfo info) => lineEnd = info.eventPosition.game;
@override
void onPanEnd(DragEndInfo info) {
if (lineEnd != null) {
final line = lineEnd! - lineStart!;
_turboChargeBall(line);
lineEnd = null;
lineStart = null;
}
}
void _turboChargeBall(Vector2 line) {
final canvas = descendants().whereType<ZCanvasComponent>().single;
final ball = Ball()..initialPosition = lineStart!;
final impulse = line * -1 * 10;
ball.add(BallTurboChargingBehavior(impulse: impulse));
canvas.add(ball);
}
}
// coverage:ignore-start
class PreviewLine extends PositionComponent with HasGameRef<DebugPinballGame> {
static final _previewLinePaint = Paint()
..color = Colors.pink
..strokeWidth = 0.4
..style = PaintingStyle.stroke;
@override
void render(Canvas canvas) {
super.render(canvas);
if (gameRef.lineEnd != null) {
canvas.drawLine(
gameRef.lineStart!.toOffset(),
gameRef.lineEnd!.toOffset(),
_previewLinePaint,
);
}
}
}
class _DebugInformation extends Component with HasGameRef<DebugPinballGame> {
@override
PositionType get positionType => PositionType.widget;
final _debugTextPaint = TextPaint(
style: const TextStyle(
color: Colors.green,
fontSize: 10,
),
);
final _debugBackgroundPaint = Paint()..color = Colors.white;
@override
void render(Canvas canvas) {
final debugText = [
'FPS: ${gameRef.fps().toStringAsFixed(1)}',
'BALLS: ${gameRef.descendants().whereType<Ball>().length}',
].join(' | ');
final height = _debugTextPaint.measureTextHeight(debugText);
final position = Vector2(0, gameRef.camera.canvasSize.y - height);
canvas.drawRect(
position & Vector2(gameRef.camera.canvasSize.x, height),
_debugBackgroundPaint,
);
_debugTextPaint.render(canvas, debugText, position);
}
}
// coverage:ignore-end
| pinball/lib/game/pinball_game.dart/0 | {
"file_path": "pinball/lib/game/pinball_game.dart",
"repo_id": "pinball",
"token_count": 4356
} | 1,152 |
export 'how_to_play_dialog.dart';
| pinball/lib/how_to_play/widgets/widgets.dart/0 | {
"file_path": "pinball/lib/how_to_play/widgets/widgets.dart",
"repo_id": "pinball",
"token_count": 15
} | 1,153 |
export 'bloc/start_game_bloc.dart';
export 'widgets/start_game_listener.dart';
| pinball/lib/start_game/start_game.dart/0 | {
"file_path": "pinball/lib/start_game/start_game.dart",
"repo_id": "pinball",
"token_count": 32
} | 1,154 |
library pinball_audio;
export 'src/pinball_audio.dart';
| pinball/packages/pinball_audio/lib/pinball_audio.dart/0 | {
"file_path": "pinball/packages/pinball_audio/lib/pinball_audio.dart",
"repo_id": "pinball",
"token_count": 21
} | 1,155 |
library pinball_components;
export 'gen/gen.dart';
export 'src/pinball_components.dart';
| pinball/packages/pinball_components/lib/pinball_components.dart/0 | {
"file_path": "pinball/packages/pinball_components/lib/pinball_components.dart",
"repo_id": "pinball",
"token_count": 33
} | 1,156 |
import 'package:flame/components.dart';
import 'package:flame/input.dart';
import 'package:flutter/material.dart';
import 'package:pinball_components/pinball_components.dart';
/// enum with the available directions for an [ArrowIcon].
enum ArrowIconDirection {
/// Left.
left,
/// Right.
right,
}
/// {@template arrow_icon}
/// A [SpriteComponent] that renders a simple arrow icon.
/// {@endtemplate}
class ArrowIcon extends SpriteComponent with Tappable, HasGameRef {
/// {@macro arrow_icon}
ArrowIcon({
required Vector2 position,
required this.direction,
required this.onTap,
}) : super(position: position);
final ArrowIconDirection direction;
final VoidCallback onTap;
@override
Future<void> onLoad() async {
anchor = Anchor.center;
final sprite = Sprite(
gameRef.images.fromCache(
direction == ArrowIconDirection.left
? Assets.images.displayArrows.arrowLeft.keyName
: Assets.images.displayArrows.arrowRight.keyName,
),
);
size = sprite.originalSize / 20;
this.sprite = sprite;
}
@override
bool onTapUp(TapUpInfo info) {
onTap();
return true;
}
}
| pinball/packages/pinball_components/lib/src/components/arrow_icon.dart/0 | {
"file_path": "pinball/packages/pinball_components/lib/src/components/arrow_icon.dart",
"repo_id": "pinball",
"token_count": 414
} | 1,157 |
export 'chrome_dino_chomping_behavior.dart';
export 'chrome_dino_mouth_opening_behavior.dart';
export 'chrome_dino_spitting_behavior.dart';
export 'chrome_dino_swiveling_behavior.dart';
| pinball/packages/pinball_components/lib/src/components/chrome_dino/behaviors/behaviors.dart/0 | {
"file_path": "pinball/packages/pinball_components/lib/src/components/chrome_dino/behaviors/behaviors.dart",
"repo_id": "pinball",
"token_count": 67
} | 1,158 |
import 'package:flame/components.dart';
import 'package:flutter/material.dart';
import 'package:pinball_components/pinball_components.dart';
import 'package:pinball_ui/pinball_ui.dart';
final _boldLabelTextPaint = TextPaint(
style: const TextStyle(
fontSize: 1.8,
color: PinballColors.white,
fontFamily: PinballFonts.pixeloidSans,
fontWeight: FontWeight.w700,
),
);
final _labelTextPaint = TextPaint(
style: const TextStyle(
fontSize: 1.8,
color: PinballColors.white,
fontFamily: PinballFonts.pixeloidSans,
fontWeight: FontWeight.w400,
),
);
/// {@template error_component}
/// A plain visual component used to show errors for the user.
/// {@endtemplate}
class ErrorComponent extends SpriteComponent with HasGameRef {
/// {@macro error_component}
ErrorComponent({required this.label, Vector2? position})
: _textPaint = _labelTextPaint,
super(
position: position,
);
/// {@macro error_component}
ErrorComponent.bold({required this.label, Vector2? position})
: _textPaint = _boldLabelTextPaint,
super(
position: position,
);
/// Text shown on the error message.
final String label;
final TextPaint _textPaint;
List<String> _splitInLines() {
final maxWidth = size.x - 8;
final lines = <String>[];
var currentLine = '';
final words = label.split(' ');
while (words.isNotEmpty) {
final word = words.removeAt(0);
if (_textPaint.measureTextWidth('$currentLine $word') <= maxWidth) {
currentLine = '$currentLine $word'.trim();
} else {
lines.add(currentLine);
currentLine = word;
}
}
lines.add(currentLine);
return lines;
}
@override
Future<void> onLoad() async {
anchor = Anchor.center;
final sprite = await gameRef.loadSprite(
Assets.images.errorBackground.keyName,
);
size = sprite.originalSize / 20;
this.sprite = sprite;
final lines = _splitInLines();
// Calculates vertical offset based on the number of lines of text to be
// displayed. This offset is used to keep the middle of the multi-line text
// at the center of the [ErrorComponent].
final yOffset = ((size.y / 2.2) / lines.length) * 1.5;
for (var i = 0; i < lines.length; i++) {
await add(
TextComponent(
position: Vector2(size.x / 2, yOffset + 2.2 * i),
size: Vector2(size.x - 4, 2.2),
text: lines[i],
textRenderer: _textPaint,
anchor: Anchor.center,
),
);
}
}
}
| pinball/packages/pinball_components/lib/src/components/error_component.dart/0 | {
"file_path": "pinball/packages/pinball_components/lib/src/components/error_component.dart",
"repo_id": "pinball",
"token_count": 1012
} | 1,159 |
export 'google_word_animating_behavior.dart';
| pinball/packages/pinball_components/lib/src/components/google_word/behaviors/behaviors.dart/0 | {
"file_path": "pinball/packages/pinball_components/lib/src/components/google_word/behaviors/behaviors.dart",
"repo_id": "pinball",
"token_count": 15
} | 1,160 |
import 'package:flame_forge2d/flame_forge2d.dart';
import 'package:pinball_components/pinball_components.dart';
import 'package:pinball_components/src/components/layer_sensor/behaviors/layer_filtering_behavior.dart';
import 'package:pinball_flame/pinball_flame.dart';
/// {@template layer_entrance_orientation}
/// Determines if a layer entrance is oriented [up] or [down] on the board.
/// {@endtemplate}
enum LayerEntranceOrientation {
/// Facing up on the Board.
up,
/// Facing down on the Board.
down,
}
/// {@template layer_sensor}
/// [BodyComponent] located at the entrance and exit of a [Layer].
///
/// By default the base [layer] is set to [Layer.board] and the
/// [outsideZIndex] is set to [ZIndexes.ballOnBoard].
/// {@endtemplate}
abstract class LayerSensor extends BodyComponent with InitialPosition, Layered {
/// {@macro layer_sensor}
LayerSensor({
required this.insideLayer,
Layer? outsideLayer,
required this.insideZIndex,
int? outsideZIndex,
required this.orientation,
}) : outsideLayer = outsideLayer ?? Layer.board,
outsideZIndex = outsideZIndex ?? ZIndexes.ballOnBoard,
super(
renderBody: false,
children: [LayerFilteringBehavior()],
) {
layer = Layer.opening;
}
final Layer insideLayer;
final Layer outsideLayer;
final int insideZIndex;
final int outsideZIndex;
/// The [Shape] of the [LayerSensor].
Shape get shape;
/// {@macro layer_entrance_orientation}
final LayerEntranceOrientation orientation;
@override
Body createBody() {
final fixtureDef = FixtureDef(
shape,
isSensor: true,
);
final bodyDef = BodyDef(position: initialPosition);
return world.createBody(bodyDef)..createFixture(fixtureDef);
}
}
| pinball/packages/pinball_components/lib/src/components/layer_sensor/layer_sensor.dart/0 | {
"file_path": "pinball/packages/pinball_components/lib/src/components/layer_sensor/layer_sensor.dart",
"repo_id": "pinball",
"token_count": 606
} | 1,161 |
part of 'plunger_cubit.dart';
enum PlungerState {
pulling,
releasing,
autoPulling,
}
extension PlungerStateX on PlungerState {
bool get isPulling =>
this == PlungerState.pulling || this == PlungerState.autoPulling;
bool get isReleasing => this == PlungerState.releasing;
bool get isAutoPulling => this == PlungerState.autoPulling;
}
| pinball/packages/pinball_components/lib/src/components/plunger/cubit/plunger_state.dart/0 | {
"file_path": "pinball/packages/pinball_components/lib/src/components/plunger/cubit/plunger_state.dart",
"repo_id": "pinball",
"token_count": 129
} | 1,162 |
import 'dart:math' as math;
import 'package:flame/components.dart';
import 'package:flame_forge2d/flame_forge2d.dart';
import 'package:pinball_components/pinball_components.dart';
import 'package:pinball_flame/pinball_flame.dart';
/// {@template spaceship_rail}
/// Rail exiting the [AndroidSpaceship].
/// {@endtemplate}
class SpaceshipRail extends Component {
/// {@macro spaceship_rail}
SpaceshipRail()
: super(
children: [
_SpaceshipRail(),
_SpaceshipRailExit(),
_SpaceshipRailExitSpriteComponent()
],
);
}
class _SpaceshipRail extends BodyComponent with Layered, ZIndex {
_SpaceshipRail()
: super(
children: [_SpaceshipRailSpriteComponent()],
renderBody: false,
) {
layer = Layer.spaceshipExitRail;
zIndex = ZIndexes.spaceshipRail;
}
List<FixtureDef> _createFixtureDefs() {
final topArcShape = ArcShape(
center: Vector2(-35.1, -30.9),
arcRadius: 2.5,
angle: math.pi,
rotation: 0.2,
);
final topLeftCurveShape = BezierCurveShape(
controlPoints: [
Vector2(-37.6, -30.4),
Vector2(-37.8, -23.9),
Vector2(-30.93, -18.2),
],
);
final middleLeftCurveShape = BezierCurveShape(
controlPoints: [
topLeftCurveShape.vertices.last,
Vector2(-22.6, -10.3),
Vector2(-29.5, -0.2),
],
);
final bottomLeftCurveShape = BezierCurveShape(
controlPoints: [
middleLeftCurveShape.vertices.last,
Vector2(-35.6, 8.6),
Vector2(-31.3, 18.3),
],
);
final topRightStraightShape = EdgeShape()
..set(
Vector2(-27.2, -21.3),
Vector2(-33, -31.3),
);
final middleRightCurveShape = BezierCurveShape(
controlPoints: [
topRightStraightShape.vertex1,
Vector2(-16.5, -11.4),
Vector2(-25.29, 1.7),
],
);
final bottomRightCurveShape = BezierCurveShape(
controlPoints: [
middleRightCurveShape.vertices.last,
Vector2(-29.91, 8.5),
Vector2(-26.8, 15.7),
],
);
return [
FixtureDef(topArcShape),
FixtureDef(topLeftCurveShape),
FixtureDef(middleLeftCurveShape),
FixtureDef(bottomLeftCurveShape),
FixtureDef(topRightStraightShape),
FixtureDef(middleRightCurveShape),
FixtureDef(bottomRightCurveShape),
];
}
@override
Body createBody() {
final body = world.createBody(BodyDef());
_createFixtureDefs().forEach(body.createFixture);
return body;
}
}
class _SpaceshipRailSpriteComponent extends SpriteComponent with HasGameRef {
_SpaceshipRailSpriteComponent()
: super(
anchor: Anchor.center,
position: Vector2(-29.4, -5.7),
);
@override
Future<void> onLoad() async {
await super.onLoad();
final sprite = Sprite(
gameRef.images.fromCache(
Assets.images.android.rail.main.keyName,
),
);
this.sprite = sprite;
size = sprite.originalSize / 10;
}
}
class _SpaceshipRailExitSpriteComponent extends SpriteComponent
with HasGameRef, ZIndex {
_SpaceshipRailExitSpriteComponent()
: super(
anchor: Anchor.center,
position: Vector2(-28, 19.4),
) {
zIndex = ZIndexes.spaceshipRailExit;
}
@override
Future<void> onLoad() async {
await super.onLoad();
final sprite = Sprite(
gameRef.images.fromCache(
Assets.images.android.rail.exit.keyName,
),
);
this.sprite = sprite;
size = sprite.originalSize / 10;
}
}
class _SpaceshipRailExit extends LayerSensor {
_SpaceshipRailExit()
: super(
orientation: LayerEntranceOrientation.down,
insideLayer: Layer.spaceshipExitRail,
insideZIndex: ZIndexes.ballOnSpaceshipRail,
) {
layer = Layer.spaceshipExitRail;
}
@override
Shape get shape {
return ArcShape(
center: Vector2(-29, 19),
arcRadius: 2.5,
angle: math.pi * 0.4,
rotation: -1.4,
);
}
}
| pinball/packages/pinball_components/lib/src/components/spaceship_rail.dart/0 | {
"file_path": "pinball/packages/pinball_components/lib/src/components/spaceship_rail.dart",
"repo_id": "pinball",
"token_count": 1826
} | 1,163 |
part of 'sparky_computer_cubit.dart';
enum SparkyComputerState {
withoutBall,
withBall,
}
| pinball/packages/pinball_components/lib/src/components/sparky_computer/cubit/sparky_computer_state.dart/0 | {
"file_path": "pinball/packages/pinball_components/lib/src/components/sparky_computer/cubit/sparky_computer_state.dart",
"repo_id": "pinball",
"token_count": 37
} | 1,164 |
import 'dart:async';
import 'package:flame/components.dart';
import 'package:flame_forge2d/flame_forge2d.dart';
import 'package:flutter/material.dart';
import 'package:pinball_components/pinball_components.dart';
extension BodyTrace on BodyComponent {
void trace({Color color = const Color(0xFFFF0000)}) {
paint = Paint()..color = color;
renderBody = true;
unawaited(
mounted.whenComplete(() {
descendants().whereType<JointAnchor>().forEach((anchor) {
final fixtureDef = FixtureDef(CircleShape()..radius = 0.5);
anchor.body.createFixture(fixtureDef);
anchor.renderBody = true;
});
}),
);
}
}
mixin Traceable on Forge2DGame {
late final bool trace;
Future<void> traceAllBodies({
Color color = const Color(0xFFFF0000),
}) async {
if (trace) {
await ready();
descendants()
.whereType<BodyComponent>()
.forEach((bodyComponent) => bodyComponent.trace());
descendants()
.whereType<HasPaint>()
.forEach((sprite) => sprite.setOpacity(0.5));
}
}
}
| pinball/packages/pinball_components/sandbox/lib/common/trace.dart/0 | {
"file_path": "pinball/packages/pinball_components/sandbox/lib/common/trace.dart",
"repo_id": "pinball",
"token_count": 451
} | 1,165 |
import 'package:flame/input.dart';
import 'package:pinball_components/pinball_components.dart';
import 'package:sandbox/stories/ball/basic_ball_game.dart';
class FlipperGame extends BallGame with KeyboardEvents {
FlipperGame()
: super(
imagesFileNames: [
Assets.images.flipper.left.keyName,
Assets.images.flipper.right.keyName,
],
);
static const description = '''
Shows how Flippers are rendered.
- Activate the "trace" parameter to overlay the body.
- Tap anywhere on the screen to spawn a ball into the game.
- Press left arrow key or "A" to move the left flipper.
- Press right arrow key or "D" to move the right flipper.
''';
late Flipper leftFlipper;
late Flipper rightFlipper;
@override
Future<void> onLoad() async {
await super.onLoad();
final center = screenToWorld(camera.viewport.canvasSize! / 2);
await addAll([
leftFlipper = Flipper(side: BoardSide.left)
..initialPosition = center - Vector2(Flipper.size.x, 0),
rightFlipper = Flipper(side: BoardSide.right)
..initialPosition = center + Vector2(Flipper.size.x, 0),
]);
await traceAllBodies();
}
}
| pinball/packages/pinball_components/sandbox/lib/stories/bottom_group/flipper_game.dart/0 | {
"file_path": "pinball/packages/pinball_components/sandbox/lib/stories/bottom_group/flipper_game.dart",
"repo_id": "pinball",
"token_count": 456
} | 1,166 |
import 'dart:async';
import 'package:flame/input.dart';
import 'package:flame_bloc/flame_bloc.dart';
import 'package:pinball_components/pinball_components.dart';
import 'package:sandbox/stories/ball/basic_ball_game.dart';
class SignpostGame extends BallGame {
SignpostGame()
: super(
imagesFileNames: [
Assets.images.signpost.inactive.keyName,
Assets.images.signpost.active1.keyName,
Assets.images.signpost.active2.keyName,
Assets.images.signpost.active3.keyName,
],
);
static const description = '''
Shows how a Signpost is rendered.
- Activate the "trace" parameter to overlay the body.
- Tap to progress the sprite.
''';
late final SignpostCubit _bloc;
@override
Future<void> onLoad() async {
await super.onLoad();
_bloc = SignpostCubit();
camera.followVector2(Vector2.zero());
await add(
FlameMultiBlocProvider(
providers: [
FlameBlocProvider<SignpostCubit, SignpostState>.value(
value: _bloc,
),
FlameBlocProvider<DashBumpersCubit, DashBumpersState>(
create: DashBumpersCubit.new,
),
],
children: [
Signpost(),
],
),
);
await traceAllBodies();
}
@override
void onTap() {
super.onTap();
_bloc.onProgressed();
}
}
| pinball/packages/pinball_components/sandbox/lib/stories/flutter_forest/signpost_game.dart/0 | {
"file_path": "pinball/packages/pinball_components/sandbox/lib/stories/flutter_forest/signpost_game.dart",
"repo_id": "pinball",
"token_count": 610
} | 1,167 |
import 'dart:async';
import 'package:flame/extensions.dart';
import 'package:pinball_components/pinball_components.dart';
import 'package:sandbox/stories/ball/basic_ball_game.dart';
class SparkyBumperGame extends BallGame {
static const description = '''
Shows how a SparkyBumper is rendered.
- Activate the "trace" parameter to overlay the body.
- Tap anywhere on the screen to spawn a ball into the game.
''';
@override
Future<void> onLoad() async {
await super.onLoad();
await images.loadAll([
Assets.images.sparky.bumper.a.lit.keyName,
Assets.images.sparky.bumper.a.dimmed.keyName,
Assets.images.sparky.bumper.b.lit.keyName,
Assets.images.sparky.bumper.b.dimmed.keyName,
Assets.images.sparky.bumper.c.lit.keyName,
Assets.images.sparky.bumper.c.dimmed.keyName,
]);
final center = screenToWorld(camera.viewport.canvasSize! / 2);
final sparkyBumperA = SparkyBumper.a()
..initialPosition = Vector2(center.x - 20, center.y + 20)
..priority = 1;
final sparkyBumperB = SparkyBumper.b()
..initialPosition = Vector2(center.x - 10, center.y - 10)
..priority = 1;
final sparkyBumperC = SparkyBumper.c()
..initialPosition = Vector2(center.x + 20, center.y)
..priority = 1;
await addAll([
sparkyBumperA,
sparkyBumperB,
sparkyBumperC,
]);
await traceAllBodies();
}
}
| pinball/packages/pinball_components/sandbox/lib/stories/sparky_scorch/sparky_bumper_game.dart/0 | {
"file_path": "pinball/packages/pinball_components/sandbox/lib/stories/sparky_scorch/sparky_bumper_game.dart",
"repo_id": "pinball",
"token_count": 572
} | 1,168 |
// ignore_for_file: cascade_invocations
import 'package:flame/components.dart';
import 'package:flame_test/flame_test.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:pinball_components/pinball_components.dart';
import 'package:pinball_components/src/components/android_animatronic/behaviors/behaviors.dart';
import '../../helpers/helpers.dart';
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
final asset = Assets.images.android.spaceship.animatronic.keyName;
final flameTester = FlameTester(() => TestGame([asset]));
group('AndroidAnimatronic', () {
flameTester.testGameWidget(
'renders correctly',
setUp: (game, tester) async {
await game.images.load(asset);
await game.ensureAdd(AndroidAnimatronic());
game.camera.followVector2(Vector2.zero());
await tester.pump();
},
verify: (game, tester) async {
final animationDuration = game
.firstChild<AndroidAnimatronic>()!
.firstChild<SpriteAnimationComponent>()!
.animation!
.totalDuration();
await expectLater(
find.byGame<TestGame>(),
matchesGoldenFile('golden/android_animatronic/start.png'),
);
game.update(animationDuration * 0.5);
await tester.pump();
await expectLater(
find.byGame<TestGame>(),
matchesGoldenFile('golden/android_animatronic/middle.png'),
);
game.update(animationDuration * 0.5);
await tester.pump();
await expectLater(
find.byGame<TestGame>(),
matchesGoldenFile('golden/android_animatronic/end.png'),
);
},
);
flameTester.test(
'loads correctly',
(game) async {
final androidAnimatronic = AndroidAnimatronic();
await game.ensureAdd(androidAnimatronic);
expect(game.contains(androidAnimatronic), isTrue);
},
);
group('adds', () {
flameTester.test('new children', (game) async {
final component = Component();
final androidAnimatronic = AndroidAnimatronic(
children: [component],
);
await game.ensureAdd(androidAnimatronic);
expect(androidAnimatronic.children, contains(component));
});
flameTester.test('a AndroidAnimatronicBallContactBehavior', (game) async {
final androidAnimatronic = AndroidAnimatronic();
await game.ensureAdd(androidAnimatronic);
expect(
androidAnimatronic.children
.whereType<AndroidAnimatronicBallContactBehavior>()
.single,
isNotNull,
);
});
});
});
}
| pinball/packages/pinball_components/test/src/components/android_animatronic_test.dart/0 | {
"file_path": "pinball/packages/pinball_components/test/src/components/android_animatronic_test.dart",
"repo_id": "pinball",
"token_count": 1130
} | 1,169 |
import 'package:bloc_test/bloc_test.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:pinball_components/pinball_components.dart';
import 'package:pinball_theme/pinball_theme.dart';
void main() {
group(
'BallCubit',
() {
blocTest<BallCubit, BallState>(
'onCharacterSelected emits new theme',
build: BallCubit.new,
act: (bloc) => bloc.onCharacterSelected(const DinoTheme()),
expect: () => [const BallState(characterTheme: DinoTheme())],
);
},
);
}
| pinball/packages/pinball_components/test/src/components/ball/cubit/ball_cubit_test.dart/0 | {
"file_path": "pinball/packages/pinball_components/test/src/components/ball/cubit/ball_cubit_test.dart",
"repo_id": "pinball",
"token_count": 216
} | 1,170 |
import 'package:bloc_test/bloc_test.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:pinball_components/pinball_components.dart';
void main() {
group('FlipperCubit', () {
test('can be instantiated', () {
expect(FlipperCubit(), isA<FlipperCubit>());
});
blocTest<FlipperCubit, FlipperState>(
'moves',
build: FlipperCubit.new,
act: (cubit) => cubit
..moveUp()
..moveDown(),
expect: () => [
FlipperState.movingUp,
FlipperState.movingDown,
],
);
});
}
| pinball/packages/pinball_components/test/src/components/flipper/cubit/flipper_cubit_test.dart/0 | {
"file_path": "pinball/packages/pinball_components/test/src/components/flipper/cubit/flipper_cubit_test.dart",
"repo_id": "pinball",
"token_count": 256
} | 1,171 |
// ignore_for_file: cascade_invocations
import 'package:flame_forge2d/flame_forge2d.dart';
import 'package:flame_test/flame_test.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:mocktail/mocktail.dart';
import 'package:pinball_components/pinball_components.dart';
import 'package:pinball_components/src/components/layer_sensor/behaviors/behaviors.dart';
import 'package:pinball_flame/pinball_flame.dart';
import '../../../../helpers/helpers.dart';
class _TestLayerSensor extends LayerSensor {
_TestLayerSensor({
required LayerEntranceOrientation orientation,
required int insideZIndex,
required Layer insideLayer,
}) : super(
insideLayer: insideLayer,
insideZIndex: insideZIndex,
orientation: orientation,
);
@override
Shape get shape => PolygonShape()..setAsBoxXY(1, 1);
}
class _MockBall extends Mock implements Ball {}
class _MockBody extends Mock implements Body {}
class _MockContact extends Mock implements Contact {}
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
final flameTester = FlameTester(TestGame.new);
group(
'LayerSensorBehavior',
() {
test('can be instantiated', () {
expect(
LayerFilteringBehavior(),
isA<LayerFilteringBehavior>(),
);
});
flameTester.test(
'loads',
(game) async {
final behavior = LayerFilteringBehavior();
final parent = _TestLayerSensor(
orientation: LayerEntranceOrientation.down,
insideZIndex: 1,
insideLayer: Layer.spaceshipEntranceRamp,
);
await parent.add(behavior);
await game.ensureAdd(parent);
expect(game.contains(parent), isTrue);
},
);
group('beginContact', () {
late Ball ball;
late Body body;
late int insideZIndex;
late Layer insideLayer;
setUp(() {
ball = _MockBall();
body = _MockBody();
insideZIndex = 1;
insideLayer = Layer.spaceshipEntranceRamp;
when(() => ball.body).thenReturn(body);
when(() => ball.layer).thenReturn(Layer.board);
});
flameTester.test(
'changes ball layer and zIndex '
'when a ball enters and exits a downward oriented LayerSensor',
(game) async {
final parent = _TestLayerSensor(
orientation: LayerEntranceOrientation.down,
insideZIndex: 1,
insideLayer: insideLayer,
)..initialPosition = Vector2(0, 10);
final behavior = LayerFilteringBehavior();
await parent.add(behavior);
await game.ensureAdd(parent);
when(() => body.linearVelocity).thenReturn(Vector2(0, -1));
behavior.beginContact(ball, _MockContact());
verify(() => ball.layer = insideLayer).called(1);
verify(() => ball.zIndex = insideZIndex).called(1);
when(() => ball.layer).thenReturn(insideLayer);
behavior.beginContact(ball, _MockContact());
verify(() => ball.layer = Layer.board);
verify(() => ball.zIndex = ZIndexes.ballOnBoard).called(1);
});
flameTester.test(
'changes ball layer and zIndex '
'when a ball enters and exits an upward oriented LayerSensor',
(game) async {
final parent = _TestLayerSensor(
orientation: LayerEntranceOrientation.up,
insideZIndex: 1,
insideLayer: insideLayer,
)..initialPosition = Vector2(0, 10);
final behavior = LayerFilteringBehavior();
await parent.add(behavior);
await game.ensureAdd(parent);
when(() => body.linearVelocity).thenReturn(Vector2(0, 1));
behavior.beginContact(ball, _MockContact());
verify(() => ball.layer = insideLayer).called(1);
verify(() => ball.zIndex = 1).called(1);
when(() => ball.layer).thenReturn(insideLayer);
behavior.beginContact(ball, _MockContact());
verify(() => ball.layer = Layer.board);
verify(() => ball.zIndex = ZIndexes.ballOnBoard).called(1);
});
});
},
);
}
| pinball/packages/pinball_components/test/src/components/layer_sensor/behavior/layer_filtering_behavior_test.dart/0 | {
"file_path": "pinball/packages/pinball_components/test/src/components/layer_sensor/behavior/layer_filtering_behavior_test.dart",
"repo_id": "pinball",
"token_count": 1811
} | 1,172 |
// ignore_for_file: cascade_invocations
import 'package:flame/components.dart';
import 'package:flame_test/flame_test.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:pinball_components/pinball_components.dart';
import '../../helpers/helpers.dart';
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
final assets = [
Assets.images.plunger.rocket.keyName,
];
final flameTester = FlameTester(() => TestGame(assets));
group('RocketSpriteComponent', () {
flameTester.testGameWidget(
'renders correctly',
setUp: (game, tester) async {
await game.images.loadAll(assets);
await game.ensureAdd(RocketSpriteComponent());
game.camera
..followVector2(Vector2.zero())
..zoom = 8;
await tester.pump();
},
verify: (game, tester) async {
await expectLater(
find.byGame<TestGame>(),
matchesGoldenFile('golden/rocket.png'),
);
},
);
});
}
| pinball/packages/pinball_components/test/src/components/rocket_test.dart/0 | {
"file_path": "pinball/packages/pinball_components/test/src/components/rocket_test.dart",
"repo_id": "pinball",
"token_count": 412
} | 1,173 |
// ignore_for_file: cascade_invocations
import 'package:flame/components.dart';
import 'package:flame_test/flame_test.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:pinball_components/pinball_components.dart';
import '../../helpers/helpers.dart';
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
group('SparkyAnimatronic', () {
final asset = Assets.images.sparky.animatronic.keyName;
final flameTester = FlameTester(() => TestGame([asset]));
flameTester.testGameWidget(
'renders correctly',
setUp: (game, tester) async {
await game.images.load(asset);
await game.ensureAdd(SparkyAnimatronic()..playing = true);
await tester.pump();
game.camera.followVector2(Vector2.zero());
},
verify: (game, tester) async {
final animationDuration =
game.firstChild<SparkyAnimatronic>()!.animation!.totalDuration();
await expectLater(
find.byGame<TestGame>(),
matchesGoldenFile('golden/sparky_animatronic/start.png'),
);
game.update(animationDuration * 0.25);
await tester.pump();
await expectLater(
find.byGame<TestGame>(),
matchesGoldenFile('golden/sparky_animatronic/middle.png'),
);
game.update(animationDuration * 0.75);
await tester.pump();
await expectLater(
find.byGame<TestGame>(),
matchesGoldenFile('golden/sparky_animatronic/end.png'),
);
},
);
flameTester.test(
'loads correctly',
(game) async {
final sparkyAnimatronic = SparkyAnimatronic();
await game.ensureAdd(sparkyAnimatronic);
expect(game.contains(sparkyAnimatronic), isTrue);
},
);
flameTester.test('adds new children', (game) async {
final component = Component();
final sparkyAnimatronic = SparkyAnimatronic(
children: [component],
);
await game.ensureAdd(sparkyAnimatronic);
expect(sparkyAnimatronic.children, contains(component));
});
});
}
| pinball/packages/pinball_components/test/src/components/sparky_animatronic_test.dart/0 | {
"file_path": "pinball/packages/pinball_components/test/src/components/sparky_animatronic_test.dart",
"repo_id": "pinball",
"token_count": 882
} | 1,174 |
import 'package:flame_forge2d/flame_forge2d.dart';
import 'package:pinball_flame/pinball_flame.dart';
/// {@template layer_contact_behavior}
/// Switches the z-index of any [ZIndex] body that contacts with it.
/// {@endtemplate}
class ZIndexContactBehavior extends ContactBehavior<BodyComponent> {
/// {@macro layer_contact_behavior}
ZIndexContactBehavior({
required int zIndex,
bool onBegin = true,
}) {
if (onBegin) {
onBeginContact = (other, _) => _changeZIndex(other, zIndex);
} else {
onEndContact = (other, _) => _changeZIndex(other, zIndex);
}
}
void _changeZIndex(Object other, int zIndex) {
if (other is! ZIndex) return;
if (other.zIndex == zIndex) return;
other.zIndex = zIndex;
}
}
| pinball/packages/pinball_flame/lib/src/behaviors/z_index_contact_behavior.dart/0 | {
"file_path": "pinball/packages/pinball_flame/lib/src/behaviors/z_index_contact_behavior.dart",
"repo_id": "pinball",
"token_count": 277
} | 1,175 |
// ignore_for_file: cascade_invocations
import 'dart:math' as math;
import 'package:flame_forge2d/flame_forge2d.dart';
import 'package:flame_test/flame_test.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:pinball_flame/pinball_flame.dart';
class _TestLayeredBodyComponent extends BodyComponent with Layered {
@override
Body createBody() {
final fixtureDef = FixtureDef(CircleShape());
return world.createBody(BodyDef())..createFixture(fixtureDef);
}
}
class _TestBodyComponent extends BodyComponent {
@override
Body createBody() {
final fixtureDef = FixtureDef(CircleShape());
return world.createBody(BodyDef())..createFixture(fixtureDef);
}
}
void main() {
final flameTester = FlameTester(Forge2DGame.new);
group('Layered', () {
void _expectLayerOnFixtures({
required List<Fixture> fixtures,
required Layer layer,
}) {
expect(fixtures.length, greaterThan(0));
for (final fixture in fixtures) {
expect(
fixture.filterData.categoryBits,
equals(layer.maskBits),
);
expect(fixture.filterData.maskBits, equals(layer.maskBits));
}
}
flameTester.test('TestBodyComponent has fixtures', (game) async {
final component = _TestBodyComponent();
await game.ensureAdd(component);
});
test('correctly sets and gets', () {
final component = _TestLayeredBodyComponent()
..layer = Layer.spaceshipEntranceRamp;
expect(component.layer, Layer.spaceshipEntranceRamp);
});
flameTester.test(
'layers correctly before being loaded',
(game) async {
const expectedLayer = Layer.spaceshipEntranceRamp;
final component = _TestLayeredBodyComponent()..layer = expectedLayer;
await game.ensureAdd(component);
_expectLayerOnFixtures(
fixtures: component.body.fixtures,
layer: expectedLayer,
);
},
);
flameTester.test(
'layers correctly before being loaded '
'when multiple different sets',
(game) async {
const expectedLayer = Layer.launcher;
final component = _TestLayeredBodyComponent()
..layer = Layer.spaceshipEntranceRamp;
expect(component.layer, isNot(equals(expectedLayer)));
component.layer = expectedLayer;
await game.ensureAdd(component);
_expectLayerOnFixtures(
fixtures: component.body.fixtures,
layer: expectedLayer,
);
},
);
flameTester.test(
'layers correctly after being loaded',
(game) async {
const expectedLayer = Layer.spaceshipEntranceRamp;
final component = _TestLayeredBodyComponent();
await game.ensureAdd(component);
component.layer = expectedLayer;
_expectLayerOnFixtures(
fixtures: component.body.fixtures,
layer: expectedLayer,
);
},
);
flameTester.test(
'layers correctly after being loaded '
'when multiple different sets',
(game) async {
const expectedLayer = Layer.launcher;
final component = _TestLayeredBodyComponent();
await game.ensureAdd(component);
component.layer = Layer.spaceshipEntranceRamp;
expect(component.layer, isNot(equals(expectedLayer)));
component.layer = expectedLayer;
_expectLayerOnFixtures(
fixtures: component.body.fixtures,
layer: expectedLayer,
);
},
);
flameTester.test(
'defaults to Layer.all '
'when no layer is given',
(game) async {
final component = _TestLayeredBodyComponent();
await game.ensureAdd(component);
expect(component.layer, equals(Layer.all));
},
);
flameTester.test(
'nested Layered children will keep their layer',
(game) async {
const parentLayer = Layer.spaceshipEntranceRamp;
const childLayer = Layer.board;
final component = _TestLayeredBodyComponent()..layer = parentLayer;
final childComponent = _TestLayeredBodyComponent()..layer = childLayer;
await component.add(childComponent);
await game.ensureAdd(component);
expect(childLayer, isNot(equals(parentLayer)));
for (final child in component.children) {
expect(
(child as _TestLayeredBodyComponent).layer,
equals(childLayer),
);
}
},
);
flameTester.test(
'nested children will keep their layer',
(game) async {
const parentLayer = Layer.spaceshipEntranceRamp;
final component = _TestLayeredBodyComponent()..layer = parentLayer;
final childComponent = _TestBodyComponent();
await component.add(childComponent);
await game.ensureAdd(component);
for (final child in component.children) {
expect(
(child as _TestBodyComponent)
.body
.fixtures
.first
.filterData
.maskBits,
equals(Filter().maskBits),
);
}
},
);
});
group('LayerMaskBits', () {
test('all types are different', () {
for (final layer in Layer.values) {
for (final otherLayer in Layer.values) {
if (layer != otherLayer) {
expect(layer.maskBits, isNot(equals(otherLayer.maskBits)));
}
}
}
});
test('all maskBits are smaller than 2^16 ', () {
final maxMaskBitSize = math.pow(2, 16);
for (final layer in Layer.values) {
expect(layer.maskBits, isNot(greaterThan(maxMaskBitSize)));
}
});
});
}
| pinball/packages/pinball_flame/test/layer_test.dart/0 | {
"file_path": "pinball/packages/pinball_flame/test/layer_test.dart",
"repo_id": "pinball",
"token_count": 2358
} | 1,176 |
import 'dart:math' as math;
import 'package:flame/extensions.dart';
import 'package:flame/game.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:pinball_flame/pinball_flame.dart';
void main() {
group('EllipseShape', () {
test('can be instantiated', () {
expect(
EllipseShape(
center: Vector2.zero(),
majorRadius: 10,
minorRadius: 8,
),
isA<EllipseShape>(),
);
});
test('rotate returns vertices rotated', () {
const rotationAngle = 2 * math.pi;
final ellipseShape = EllipseShape(
center: Vector2.zero(),
majorRadius: 10,
minorRadius: 8,
);
final ellipseShapeRotated = EllipseShape(
center: Vector2.zero(),
majorRadius: 10,
minorRadius: 8,
)..rotate(rotationAngle);
for (var index = 0; index < ellipseShape.vertices.length; index++) {
expect(
ellipseShape.vertices[index]..rotate(rotationAngle),
equals(ellipseShapeRotated.vertices[index]),
);
}
});
});
}
| pinball/packages/pinball_flame/test/src/shapes/ellipse_shape_test.dart/0 | {
"file_path": "pinball/packages/pinball_flame/test/src/shapes/ellipse_shape_test.dart",
"repo_id": "pinball",
"token_count": 504
} | 1,177 |
import 'package:pinball_theme/pinball_theme.dart';
/// {@template dash_theme}
/// Defines Dash character theme assets and attributes.
/// {@endtemplate}
class DashTheme extends CharacterTheme {
/// {@macro dash_theme}
const DashTheme();
@override
String get name => 'Dash';
@override
AssetGenImage get ball => Assets.images.dash.ball;
@override
AssetGenImage get background => Assets.images.dash.background;
@override
AssetGenImage get icon => Assets.images.dash.icon;
@override
AssetGenImage get leaderboardIcon => Assets.images.dash.leaderboardIcon;
@override
AssetGenImage get animation => Assets.images.dash.animation;
}
| pinball/packages/pinball_theme/lib/src/themes/dash_theme.dart/0 | {
"file_path": "pinball/packages/pinball_theme/lib/src/themes/dash_theme.dart",
"repo_id": "pinball",
"token_count": 197
} | 1,178 |
export 'pinball_colors.dart';
export 'pinball_text_style.dart';
export 'pinball_theme.dart';
| pinball/packages/pinball_ui/lib/src/theme/theme.dart/0 | {
"file_path": "pinball/packages/pinball_ui/lib/src/theme/theme.dart",
"repo_id": "pinball",
"token_count": 36
} | 1,179 |
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:pinball_ui/pinball_ui.dart';
void main() {
group('PinballButton', () {
testWidgets('renders the given text and responds to taps', (tester) async {
var wasTapped = false;
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Center(
child: PinballButton(
text: 'test',
onTap: () {
wasTapped = true;
},
),
),
),
),
);
await tester.tap(find.text('test'));
expect(wasTapped, isTrue);
});
});
}
| pinball/packages/pinball_ui/test/src/widgets/pinball_button_test.dart/0 | {
"file_path": "pinball/packages/pinball_ui/test/src/widgets/pinball_button_test.dart",
"repo_id": "pinball",
"token_count": 361
} | 1,180 |
import 'package:share_repository/share_repository.dart';
/// {@template share_repository}
/// Repository to facilitate sharing scores.
/// {@endtemplate}
class ShareRepository {
/// {@macro share_repository}
const ShareRepository({
required String appUrl,
}) : _appUrl = appUrl;
final String _appUrl;
/// Url to the Github Open Source Pinball project.
static const openSourceCode = 'https://github.com/flutter/pinball';
/// Url to the Google IO Event.
static const googleIOEvent = 'https://events.google.com/io/';
/// Url to the Pinball game.
static const pinballGameUrl = 'https://pinball.flutter.dev';
/// Returns a url to share the [value] on the given [platform].
///
/// The returned url can be opened using the [url_launcher](https://pub.dev/packages/url_launcher) package.
String shareText({
required String value,
required SharePlatform platform,
}) {
final encodedUrl = Uri.encodeComponent(_appUrl);
final encodedShareText = Uri.encodeComponent(value);
switch (platform) {
case SharePlatform.twitter:
return 'https://twitter.com/intent/tweet?url=$encodedUrl&text=$encodedShareText';
case SharePlatform.facebook:
return 'https://www.facebook.com/sharer.php?u=$encodedUrl"e=$encodedShareText';
}
}
}
| pinball/packages/share_repository/lib/src/share_repository.dart/0 | {
"file_path": "pinball/packages/share_repository/lib/src/share_repository.dart",
"repo_id": "pinball",
"token_count": 424
} | 1,181 |
// ignore_for_file: prefer_const_constructors
import 'package:flutter_test/flutter_test.dart';
import 'package:pinball/game/components/backbox/bloc/backbox_bloc.dart';
import 'package:pinball_theme/pinball_theme.dart';
void main() {
group('BackboxEvent', () {
group('PlayerInitialsRequested', () {
test('can be instantiated', () {
expect(
PlayerInitialsRequested(score: 0, character: AndroidTheme()),
isNotNull,
);
});
test('supports value comparison', () {
expect(
PlayerInitialsRequested(score: 0, character: AndroidTheme()),
equals(
PlayerInitialsRequested(score: 0, character: AndroidTheme()),
),
);
expect(
PlayerInitialsRequested(score: 0, character: AndroidTheme()),
isNot(
equals(
PlayerInitialsRequested(score: 1, character: AndroidTheme()),
),
),
);
expect(
PlayerInitialsRequested(score: 0, character: AndroidTheme()),
isNot(
equals(
PlayerInitialsRequested(score: 0, character: SparkyTheme()),
),
),
);
});
});
group('PlayerInitialsSubmitted', () {
test('can be instantiated', () {
expect(
PlayerInitialsSubmitted(
score: 0,
initials: 'AAA',
character: AndroidTheme(),
),
isNotNull,
);
});
test('supports value comparison', () {
expect(
PlayerInitialsSubmitted(
score: 0,
initials: 'AAA',
character: AndroidTheme(),
),
equals(
PlayerInitialsSubmitted(
score: 0,
initials: 'AAA',
character: AndroidTheme(),
),
),
);
expect(
PlayerInitialsSubmitted(
score: 0,
initials: 'AAA',
character: AndroidTheme(),
),
isNot(
equals(
PlayerInitialsSubmitted(
score: 1,
initials: 'AAA',
character: AndroidTheme(),
),
),
),
);
expect(
PlayerInitialsSubmitted(
score: 0,
initials: 'AAA',
character: AndroidTheme(),
),
isNot(
equals(
PlayerInitialsSubmitted(
score: 0,
initials: 'AAA',
character: SparkyTheme(),
),
),
),
);
expect(
PlayerInitialsSubmitted(
score: 0,
initials: 'AAA',
character: AndroidTheme(),
),
isNot(
equals(
PlayerInitialsSubmitted(
score: 0,
initials: 'BBB',
character: AndroidTheme(),
),
),
),
);
});
});
group('ScoreShareRequested', () {
test('can be instantiated', () {
expect(
ShareScoreRequested(score: 0),
isNotNull,
);
});
test('supports value comparison', () {
expect(
ShareScoreRequested(score: 0),
equals(
ShareScoreRequested(score: 0),
),
);
expect(
ShareScoreRequested(score: 0),
isNot(
equals(
ShareScoreRequested(score: 1),
),
),
);
});
});
group('LeaderboardRequested', () {
test('can be instantiated', () {
expect(LeaderboardRequested(), isNotNull);
});
test('supports value comparison', () {
expect(LeaderboardRequested(), equals(LeaderboardRequested()));
});
});
});
}
| pinball/test/game/components/backbox/bloc/backbox_event_test.dart/0 | {
"file_path": "pinball/test/game/components/backbox/bloc/backbox_event_test.dart",
"repo_id": "pinball",
"token_count": 2096
} | 1,182 |
// ignore_for_file: cascade_invocations
import 'package:flame_bloc/flame_bloc.dart';
import 'package:flame_forge2d/flame_forge2d.dart';
import 'package:flame_test/flame_test.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:mocktail/mocktail.dart';
import 'package:pinball/game/behaviors/behaviors.dart';
import 'package:pinball/game/game.dart';
import 'package:pinball_audio/pinball_audio.dart';
import 'package:pinball_components/pinball_components.dart';
import 'package:pinball_flame/pinball_flame.dart';
class _TestGame extends Forge2DGame {
@override
Future<void> onLoad() async {
images.prefix = '';
await images.loadAll([
Assets.images.dash.bumper.main.active.keyName,
Assets.images.dash.bumper.main.inactive.keyName,
Assets.images.dash.bumper.a.active.keyName,
Assets.images.dash.bumper.a.inactive.keyName,
Assets.images.dash.bumper.b.active.keyName,
Assets.images.dash.bumper.b.inactive.keyName,
Assets.images.dash.animatronic.keyName,
Assets.images.signpost.inactive.keyName,
Assets.images.signpost.active1.keyName,
Assets.images.signpost.active2.keyName,
Assets.images.signpost.active3.keyName,
]);
}
Future<void> pump(FlutterForest child) async {
await ensureAdd(
FlameBlocProvider<GameBloc, GameState>.value(
value: _MockGameBloc(),
children: [
FlameProvider.value(
_MockPinballAudioPlayer(),
children: [
ZCanvasComponent(children: [child]),
],
),
],
),
);
}
}
class _MockPinballAudioPlayer extends Mock implements PinballAudioPlayer {}
class _MockGameBloc extends Mock implements GameBloc {}
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
final flameTester = FlameTester(_TestGame.new);
group('FlutterForest', () {
flameTester.test(
'loads correctly',
(game) async {
final component = FlutterForest();
await game.pump(component);
expect(game.descendants(), contains(component));
},
);
group('loads', () {
flameTester.test(
'a Signpost',
(game) async {
final component = FlutterForest();
await game.pump(component);
expect(
game.descendants().whereType<Signpost>().length,
equals(1),
);
},
);
flameTester.test(
'a DashAnimatronic',
(game) async {
final component = FlutterForest();
await game.pump(component);
expect(
game.descendants().whereType<DashAnimatronic>().length,
equals(1),
);
},
);
flameTester.test(
'three DashBumper',
(game) async {
final component = FlutterForest();
await game.pump(component);
expect(
game.descendants().whereType<DashBumper>().length,
equals(3),
);
},
);
flameTester.test(
'three DashBumpers with BumperNoiseBehavior',
(game) async {
final component = FlutterForest();
await game.pump(component);
final bumpers = game.descendants().whereType<DashBumper>();
for (final bumper in bumpers) {
expect(
bumper.firstChild<BumperNoiseBehavior>(),
isNotNull,
);
}
},
);
});
});
}
| pinball/test/game/components/flutter_forest/flutter_forest_test.dart/0 | {
"file_path": "pinball/test/game/components/flutter_forest/flutter_forest_test.dart",
"repo_id": "pinball",
"token_count": 1563
} | 1,183 |
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_localizations/flutter_localizations.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:mocktail/mocktail.dart';
import 'package:pinball/game/game.dart';
import 'package:pinball/l10n/l10n.dart';
import 'package:pinball_flame/pinball_flame.dart';
import 'package:pinball_ui/pinball_ui.dart';
class _MockPinballGame extends Mock implements PinballGame {}
extension _WidgetTesterX on WidgetTester {
Future<void> pumpMobileControls(PinballGame game) async {
await pumpWidget(
MaterialApp(
localizationsDelegates: const [
AppLocalizations.delegate,
GlobalMaterialLocalizations.delegate,
],
home: Scaffold(
body: MobileControls(game: game),
),
),
);
}
}
extension _CommonFindersX on CommonFinders {
Finder byPinballDpadDirection(PinballDpadDirection direction) {
return byWidgetPredicate((widget) {
return widget is PinballDpadButton && widget.direction == direction;
});
}
}
void main() {
group('MobileControls', () {
testWidgets('renders', (tester) async {
await tester.pumpMobileControls(_MockPinballGame());
expect(find.byType(PinballButton), findsOneWidget);
expect(find.byType(MobileDpad), findsOneWidget);
});
testWidgets('correctly triggers the arrow up', (tester) async {
var pressed = false;
final component = KeyboardInputController(
keyUp: {
LogicalKeyboardKey.arrowUp: () => pressed = true,
},
);
final game = _MockPinballGame();
when(game.descendants).thenReturn([component]);
await tester.pumpMobileControls(game);
await tester.tap(find.byPinballDpadDirection(PinballDpadDirection.up));
await tester.pump();
expect(pressed, isTrue);
});
testWidgets('correctly triggers the arrow down', (tester) async {
var pressed = false;
final component = KeyboardInputController(
keyUp: {
LogicalKeyboardKey.arrowDown: () => pressed = true,
},
);
final game = _MockPinballGame();
when(game.descendants).thenReturn([component]);
await tester.pumpMobileControls(game);
await tester.tap(find.byPinballDpadDirection(PinballDpadDirection.down));
await tester.pump();
expect(pressed, isTrue);
});
testWidgets('correctly triggers the arrow right', (tester) async {
var pressed = false;
final component = KeyboardInputController(
keyUp: {
LogicalKeyboardKey.arrowRight: () => pressed = true,
},
);
final game = _MockPinballGame();
when(game.descendants).thenReturn([component]);
await tester.pumpMobileControls(game);
await tester.tap(find.byPinballDpadDirection(PinballDpadDirection.right));
await tester.pump();
expect(pressed, isTrue);
});
testWidgets('correctly triggers the arrow left', (tester) async {
var pressed = false;
final component = KeyboardInputController(
keyUp: {
LogicalKeyboardKey.arrowLeft: () => pressed = true,
},
);
final game = _MockPinballGame();
when(game.descendants).thenReturn([component]);
await tester.pumpMobileControls(game);
await tester.tap(find.byPinballDpadDirection(PinballDpadDirection.left));
await tester.pump();
expect(pressed, isTrue);
});
testWidgets('correctly triggers the enter', (tester) async {
var pressed = false;
final component = KeyboardInputController(
keyUp: {
LogicalKeyboardKey.enter: () => pressed = true,
},
);
final game = _MockPinballGame();
when(game.descendants).thenReturn([component]);
await tester.pumpMobileControls(game);
await tester.tap(find.byType(PinballButton));
await tester.pump();
expect(pressed, isTrue);
});
});
}
| pinball/test/game/view/widgets/mobile_controls_test.dart/0 | {
"file_path": "pinball/test/game/view/widgets/mobile_controls_test.dart",
"repo_id": "pinball",
"token_count": 1572
} | 1,184 |
// ignore_for_file: prefer_const_constructors
import 'package:flutter_test/flutter_test.dart';
import 'package:pinball/start_game/bloc/start_game_bloc.dart';
void main() {
group('StartGameEvent', () {
test('PlayTapped supports value equality', () {
expect(
PlayTapped(),
equals(PlayTapped()),
);
});
test('ReplayTapped supports value equality', () {
expect(
ReplayTapped(),
equals(ReplayTapped()),
);
});
test('CharacterSelected supports value equality', () {
expect(
CharacterSelected(),
equals(CharacterSelected()),
);
});
test('HowToPlayFinished supports value equality', () {
expect(
HowToPlayFinished(),
equals(HowToPlayFinished()),
);
});
});
}
| pinball/test/start_game/bloc/start_game_event_test.dart/0 | {
"file_path": "pinball/test/start_game/bloc/start_game_event_test.dart",
"repo_id": "pinball",
"token_count": 335
} | 1,185 |
7048ed95a5ad3e43d697e0c397464193991fc230
| plugins/.ci/flutter_stable.version/0 | {
"file_path": "plugins/.ci/flutter_stable.version",
"repo_id": "plugins",
"token_count": 24
} | 1,186 |
rootProject.name = 'camera_android'
| plugins/packages/camera/camera_android/android/settings.gradle/0 | {
"file_path": "plugins/packages/camera/camera_android/android/settings.gradle",
"repo_id": "plugins",
"token_count": 11
} | 1,187 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.camera.features;
import android.app.Activity;
import io.flutter.plugins.camera.CameraProperties;
import io.flutter.plugins.camera.DartMessenger;
import io.flutter.plugins.camera.features.autofocus.AutoFocusFeature;
import io.flutter.plugins.camera.features.exposurelock.ExposureLockFeature;
import io.flutter.plugins.camera.features.exposureoffset.ExposureOffsetFeature;
import io.flutter.plugins.camera.features.exposurepoint.ExposurePointFeature;
import io.flutter.plugins.camera.features.flash.FlashFeature;
import io.flutter.plugins.camera.features.focuspoint.FocusPointFeature;
import io.flutter.plugins.camera.features.fpsrange.FpsRangeFeature;
import io.flutter.plugins.camera.features.noisereduction.NoiseReductionFeature;
import io.flutter.plugins.camera.features.resolution.ResolutionFeature;
import io.flutter.plugins.camera.features.resolution.ResolutionPreset;
import io.flutter.plugins.camera.features.sensororientation.SensorOrientationFeature;
import io.flutter.plugins.camera.features.zoomlevel.ZoomLevelFeature;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
/**
* These are all of our available features in the camera. Used in the Camera to access all features
* in a simpler way.
*/
public class CameraFeatures {
private static final String AUTO_FOCUS = "AUTO_FOCUS";
private static final String EXPOSURE_LOCK = "EXPOSURE_LOCK";
private static final String EXPOSURE_OFFSET = "EXPOSURE_OFFSET";
private static final String EXPOSURE_POINT = "EXPOSURE_POINT";
private static final String FLASH = "FLASH";
private static final String FOCUS_POINT = "FOCUS_POINT";
private static final String FPS_RANGE = "FPS_RANGE";
private static final String NOISE_REDUCTION = "NOISE_REDUCTION";
private static final String REGION_BOUNDARIES = "REGION_BOUNDARIES";
private static final String RESOLUTION = "RESOLUTION";
private static final String SENSOR_ORIENTATION = "SENSOR_ORIENTATION";
private static final String ZOOM_LEVEL = "ZOOM_LEVEL";
public static CameraFeatures init(
CameraFeatureFactory cameraFeatureFactory,
CameraProperties cameraProperties,
Activity activity,
DartMessenger dartMessenger,
ResolutionPreset resolutionPreset) {
CameraFeatures cameraFeatures = new CameraFeatures();
cameraFeatures.setAutoFocus(
cameraFeatureFactory.createAutoFocusFeature(cameraProperties, false));
cameraFeatures.setExposureLock(
cameraFeatureFactory.createExposureLockFeature(cameraProperties));
cameraFeatures.setExposureOffset(
cameraFeatureFactory.createExposureOffsetFeature(cameraProperties));
SensorOrientationFeature sensorOrientationFeature =
cameraFeatureFactory.createSensorOrientationFeature(
cameraProperties, activity, dartMessenger);
cameraFeatures.setSensorOrientation(sensorOrientationFeature);
cameraFeatures.setExposurePoint(
cameraFeatureFactory.createExposurePointFeature(
cameraProperties, sensorOrientationFeature));
cameraFeatures.setFlash(cameraFeatureFactory.createFlashFeature(cameraProperties));
cameraFeatures.setFocusPoint(
cameraFeatureFactory.createFocusPointFeature(cameraProperties, sensorOrientationFeature));
cameraFeatures.setFpsRange(cameraFeatureFactory.createFpsRangeFeature(cameraProperties));
cameraFeatures.setNoiseReduction(
cameraFeatureFactory.createNoiseReductionFeature(cameraProperties));
cameraFeatures.setResolution(
cameraFeatureFactory.createResolutionFeature(
cameraProperties, resolutionPreset, cameraProperties.getCameraName()));
cameraFeatures.setZoomLevel(cameraFeatureFactory.createZoomLevelFeature(cameraProperties));
return cameraFeatures;
}
private Map<String, CameraFeature> featureMap = new HashMap<>();
/**
* Gets a collection of all features that have been set.
*
* @return A collection of all features that have been set.
*/
public Collection<CameraFeature> getAllFeatures() {
return this.featureMap.values();
}
/**
* Gets the auto focus feature if it has been set.
*
* @return the auto focus feature.
*/
public AutoFocusFeature getAutoFocus() {
return (AutoFocusFeature) featureMap.get(AUTO_FOCUS);
}
/**
* Sets the instance of the auto focus feature.
*
* @param autoFocus the {@link AutoFocusFeature} instance to set.
*/
public void setAutoFocus(AutoFocusFeature autoFocus) {
this.featureMap.put(AUTO_FOCUS, autoFocus);
}
/**
* Gets the exposure lock feature if it has been set.
*
* @return the exposure lock feature.
*/
public ExposureLockFeature getExposureLock() {
return (ExposureLockFeature) featureMap.get(EXPOSURE_LOCK);
}
/**
* Sets the instance of the exposure lock feature.
*
* @param exposureLock the {@link ExposureLockFeature} instance to set.
*/
public void setExposureLock(ExposureLockFeature exposureLock) {
this.featureMap.put(EXPOSURE_LOCK, exposureLock);
}
/**
* Gets the exposure offset feature if it has been set.
*
* @return the exposure offset feature.
*/
public ExposureOffsetFeature getExposureOffset() {
return (ExposureOffsetFeature) featureMap.get(EXPOSURE_OFFSET);
}
/**
* Sets the instance of the exposure offset feature.
*
* @param exposureOffset the {@link ExposureOffsetFeature} instance to set.
*/
public void setExposureOffset(ExposureOffsetFeature exposureOffset) {
this.featureMap.put(EXPOSURE_OFFSET, exposureOffset);
}
/**
* Gets the exposure point feature if it has been set.
*
* @return the exposure point feature.
*/
public ExposurePointFeature getExposurePoint() {
return (ExposurePointFeature) featureMap.get(EXPOSURE_POINT);
}
/**
* Sets the instance of the exposure point feature.
*
* @param exposurePoint the {@link ExposurePointFeature} instance to set.
*/
public void setExposurePoint(ExposurePointFeature exposurePoint) {
this.featureMap.put(EXPOSURE_POINT, exposurePoint);
}
/**
* Gets the flash feature if it has been set.
*
* @return the flash feature.
*/
public FlashFeature getFlash() {
return (FlashFeature) featureMap.get(FLASH);
}
/**
* Sets the instance of the flash feature.
*
* @param flash the {@link FlashFeature} instance to set.
*/
public void setFlash(FlashFeature flash) {
this.featureMap.put(FLASH, flash);
}
/**
* Gets the focus point feature if it has been set.
*
* @return the focus point feature.
*/
public FocusPointFeature getFocusPoint() {
return (FocusPointFeature) featureMap.get(FOCUS_POINT);
}
/**
* Sets the instance of the focus point feature.
*
* @param focusPoint the {@link FocusPointFeature} instance to set.
*/
public void setFocusPoint(FocusPointFeature focusPoint) {
this.featureMap.put(FOCUS_POINT, focusPoint);
}
/**
* Gets the fps range feature if it has been set.
*
* @return the fps range feature.
*/
public FpsRangeFeature getFpsRange() {
return (FpsRangeFeature) featureMap.get(FPS_RANGE);
}
/**
* Sets the instance of the fps range feature.
*
* @param fpsRange the {@link FpsRangeFeature} instance to set.
*/
public void setFpsRange(FpsRangeFeature fpsRange) {
this.featureMap.put(FPS_RANGE, fpsRange);
}
/**
* Gets the noise reduction feature if it has been set.
*
* @return the noise reduction feature.
*/
public NoiseReductionFeature getNoiseReduction() {
return (NoiseReductionFeature) featureMap.get(NOISE_REDUCTION);
}
/**
* Sets the instance of the noise reduction feature.
*
* @param noiseReduction the {@link NoiseReductionFeature} instance to set.
*/
public void setNoiseReduction(NoiseReductionFeature noiseReduction) {
this.featureMap.put(NOISE_REDUCTION, noiseReduction);
}
/**
* Gets the resolution feature if it has been set.
*
* @return the resolution feature.
*/
public ResolutionFeature getResolution() {
return (ResolutionFeature) featureMap.get(RESOLUTION);
}
/**
* Sets the instance of the resolution feature.
*
* @param resolution the {@link ResolutionFeature} instance to set.
*/
public void setResolution(ResolutionFeature resolution) {
this.featureMap.put(RESOLUTION, resolution);
}
/**
* Gets the sensor orientation feature if it has been set.
*
* @return the sensor orientation feature.
*/
public SensorOrientationFeature getSensorOrientation() {
return (SensorOrientationFeature) featureMap.get(SENSOR_ORIENTATION);
}
/**
* Sets the instance of the sensor orientation feature.
*
* @param sensorOrientation the {@link SensorOrientationFeature} instance to set.
*/
public void setSensorOrientation(SensorOrientationFeature sensorOrientation) {
this.featureMap.put(SENSOR_ORIENTATION, sensorOrientation);
}
/**
* Gets the zoom level feature if it has been set.
*
* @return the zoom level feature.
*/
public ZoomLevelFeature getZoomLevel() {
return (ZoomLevelFeature) featureMap.get(ZOOM_LEVEL);
}
/**
* Sets the instance of the zoom level feature.
*
* @param zoomLevel the {@link ZoomLevelFeature} instance to set.
*/
public void setZoomLevel(ZoomLevelFeature zoomLevel) {
this.featureMap.put(ZOOM_LEVEL, zoomLevel);
}
}
| plugins/packages/camera/camera_android/android/src/main/java/io/flutter/plugins/camera/features/CameraFeatures.java/0 | {
"file_path": "plugins/packages/camera/camera_android/android/src/main/java/io/flutter/plugins/camera/features/CameraFeatures.java",
"repo_id": "plugins",
"token_count": 3004
} | 1,188 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.camera;
import static org.junit.Assert.assertTrue;
import static org.mockito.ArgumentMatchers.any;
import static org.mockito.ArgumentMatchers.anyInt;
import static org.mockito.Mockito.mock;
import static org.mockito.Mockito.mockStatic;
import static org.mockito.Mockito.when;
import android.hardware.camera2.params.MeteringRectangle;
import android.util.Size;
import io.flutter.embedding.engine.systemchannels.PlatformChannel;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import org.mockito.MockedStatic;
import org.mockito.invocation.InvocationOnMock;
import org.mockito.stubbing.Answer;
public class CameraRegionUtils_convertPointToMeteringRectangleTest {
private MockedStatic<CameraRegionUtils.MeteringRectangleFactory> mockedMeteringRectangleFactory;
private Size mockCameraBoundaries;
@Before
public void setUp() {
this.mockCameraBoundaries = mock(Size.class);
when(this.mockCameraBoundaries.getWidth()).thenReturn(100);
when(this.mockCameraBoundaries.getHeight()).thenReturn(100);
mockedMeteringRectangleFactory = mockStatic(CameraRegionUtils.MeteringRectangleFactory.class);
mockedMeteringRectangleFactory
.when(
() ->
CameraRegionUtils.MeteringRectangleFactory.create(
anyInt(), anyInt(), anyInt(), anyInt(), anyInt()))
.thenAnswer(
new Answer<MeteringRectangle>() {
@Override
public MeteringRectangle answer(InvocationOnMock createInvocation) throws Throwable {
MeteringRectangle mockMeteringRectangle = mock(MeteringRectangle.class);
when(mockMeteringRectangle.getX()).thenReturn(createInvocation.getArgument(0));
when(mockMeteringRectangle.getY()).thenReturn(createInvocation.getArgument(1));
when(mockMeteringRectangle.getWidth()).thenReturn(createInvocation.getArgument(2));
when(mockMeteringRectangle.getHeight()).thenReturn(createInvocation.getArgument(3));
when(mockMeteringRectangle.getMeteringWeight())
.thenReturn(createInvocation.getArgument(4));
when(mockMeteringRectangle.equals(any()))
.thenAnswer(
new Answer<Boolean>() {
@Override
public Boolean answer(InvocationOnMock equalsInvocation)
throws Throwable {
MeteringRectangle otherMockMeteringRectangle =
equalsInvocation.getArgument(0);
return mockMeteringRectangle.getX() == otherMockMeteringRectangle.getX()
&& mockMeteringRectangle.getY() == otherMockMeteringRectangle.getY()
&& mockMeteringRectangle.getWidth()
== otherMockMeteringRectangle.getWidth()
&& mockMeteringRectangle.getHeight()
== otherMockMeteringRectangle.getHeight()
&& mockMeteringRectangle.getMeteringWeight()
== otherMockMeteringRectangle.getMeteringWeight();
}
});
return mockMeteringRectangle;
}
});
}
@After
public void tearDown() {
mockedMeteringRectangleFactory.close();
}
@Test
public void convertPointToMeteringRectangle_shouldReturnValidMeteringRectangleForCenterCoord() {
MeteringRectangle r =
CameraRegionUtils.convertPointToMeteringRectangle(
this.mockCameraBoundaries, 0.5, 0.5, PlatformChannel.DeviceOrientation.LANDSCAPE_LEFT);
assertTrue(CameraRegionUtils.MeteringRectangleFactory.create(45, 45, 10, 10, 1).equals(r));
}
@Test
public void convertPointToMeteringRectangle_shouldReturnValidMeteringRectangleForTopLeftCoord() {
MeteringRectangle r =
CameraRegionUtils.convertPointToMeteringRectangle(
this.mockCameraBoundaries, 0, 0, PlatformChannel.DeviceOrientation.LANDSCAPE_LEFT);
assertTrue(CameraRegionUtils.MeteringRectangleFactory.create(0, 0, 10, 10, 1).equals(r));
}
@Test
public void convertPointToMeteringRectangle_ShouldReturnValidMeteringRectangleForTopRightCoord() {
MeteringRectangle r =
CameraRegionUtils.convertPointToMeteringRectangle(
this.mockCameraBoundaries, 1, 0, PlatformChannel.DeviceOrientation.LANDSCAPE_LEFT);
assertTrue(CameraRegionUtils.MeteringRectangleFactory.create(89, 0, 10, 10, 1).equals(r));
}
@Test
public void
convertPointToMeteringRectangle_shouldReturnValidMeteringRectangleForBottomLeftCoord() {
MeteringRectangle r =
CameraRegionUtils.convertPointToMeteringRectangle(
this.mockCameraBoundaries, 0, 1, PlatformChannel.DeviceOrientation.LANDSCAPE_LEFT);
assertTrue(CameraRegionUtils.MeteringRectangleFactory.create(0, 89, 10, 10, 1).equals(r));
}
@Test
public void
convertPointToMeteringRectangle_shouldReturnValidMeteringRectangleForBottomRightCoord() {
MeteringRectangle r =
CameraRegionUtils.convertPointToMeteringRectangle(
this.mockCameraBoundaries, 1, 1, PlatformChannel.DeviceOrientation.LANDSCAPE_LEFT);
assertTrue(CameraRegionUtils.MeteringRectangleFactory.create(89, 89, 10, 10, 1).equals(r));
}
@Test(expected = AssertionError.class)
public void convertPointToMeteringRectangle_shouldThrowForXUpperBound() {
CameraRegionUtils.convertPointToMeteringRectangle(
this.mockCameraBoundaries, 1.5, 0, PlatformChannel.DeviceOrientation.PORTRAIT_UP);
}
@Test(expected = AssertionError.class)
public void convertPointToMeteringRectangle_shouldThrowForXLowerBound() {
CameraRegionUtils.convertPointToMeteringRectangle(
this.mockCameraBoundaries, -0.5, 0, PlatformChannel.DeviceOrientation.PORTRAIT_UP);
}
@Test(expected = AssertionError.class)
public void convertPointToMeteringRectangle_shouldThrowForYUpperBound() {
CameraRegionUtils.convertPointToMeteringRectangle(
this.mockCameraBoundaries, 0, 1.5, PlatformChannel.DeviceOrientation.PORTRAIT_UP);
}
@Test(expected = AssertionError.class)
public void convertPointToMeteringRectangle_shouldThrowForYLowerBound() {
CameraRegionUtils.convertPointToMeteringRectangle(
this.mockCameraBoundaries, 0, -0.5, PlatformChannel.DeviceOrientation.PORTRAIT_UP);
}
@Test()
public void
convertPointToMeteringRectangle_shouldRotateMeteringRectangleAccordingToUiOrientationForPortraitUp() {
MeteringRectangle r =
CameraRegionUtils.convertPointToMeteringRectangle(
this.mockCameraBoundaries, 1, 1, PlatformChannel.DeviceOrientation.PORTRAIT_UP);
assertTrue(CameraRegionUtils.MeteringRectangleFactory.create(89, 0, 10, 10, 1).equals(r));
}
@Test()
public void
convertPointToMeteringRectangle_shouldRotateMeteringRectangleAccordingToUiOrientationForPortraitDown() {
MeteringRectangle r =
CameraRegionUtils.convertPointToMeteringRectangle(
this.mockCameraBoundaries, 1, 1, PlatformChannel.DeviceOrientation.PORTRAIT_DOWN);
assertTrue(CameraRegionUtils.MeteringRectangleFactory.create(0, 89, 10, 10, 1).equals(r));
}
@Test()
public void
convertPointToMeteringRectangle_shouldRotateMeteringRectangleAccordingToUiOrientationForLandscapeLeft() {
MeteringRectangle r =
CameraRegionUtils.convertPointToMeteringRectangle(
this.mockCameraBoundaries, 1, 1, PlatformChannel.DeviceOrientation.LANDSCAPE_LEFT);
assertTrue(CameraRegionUtils.MeteringRectangleFactory.create(89, 89, 10, 10, 1).equals(r));
}
@Test()
public void
convertPointToMeteringRectangle_shouldRotateMeteringRectangleAccordingToUiOrientationForLandscapeRight() {
MeteringRectangle r =
CameraRegionUtils.convertPointToMeteringRectangle(
this.mockCameraBoundaries, 1, 1, PlatformChannel.DeviceOrientation.LANDSCAPE_RIGHT);
assertTrue(CameraRegionUtils.MeteringRectangleFactory.create(0, 0, 10, 10, 1).equals(r));
}
@Test(expected = AssertionError.class)
public void convertPointToMeteringRectangle_shouldThrowFor0WidthBoundary() {
Size mockCameraBoundaries = mock(Size.class);
when(mockCameraBoundaries.getWidth()).thenReturn(0);
when(mockCameraBoundaries.getHeight()).thenReturn(50);
CameraRegionUtils.convertPointToMeteringRectangle(
mockCameraBoundaries, 0, -0.5, PlatformChannel.DeviceOrientation.PORTRAIT_UP);
}
@Test(expected = AssertionError.class)
public void convertPointToMeteringRectangle_shouldThrowFor0HeightBoundary() {
Size mockCameraBoundaries = mock(Size.class);
when(mockCameraBoundaries.getWidth()).thenReturn(50);
when(mockCameraBoundaries.getHeight()).thenReturn(0);
CameraRegionUtils.convertPointToMeteringRectangle(
this.mockCameraBoundaries, 0, -0.5, PlatformChannel.DeviceOrientation.PORTRAIT_UP);
}
}
| plugins/packages/camera/camera_android/android/src/test/java/io/flutter/plugins/camera/CameraRegionUtils_convertPointToMeteringRectangleTest.java/0 | {
"file_path": "plugins/packages/camera/camera_android/android/src/test/java/io/flutter/plugins/camera/CameraRegionUtils_convertPointToMeteringRectangleTest.java",
"repo_id": "plugins",
"token_count": 3644
} | 1,189 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.camera.features.fpsrange;
import static org.junit.Assert.assertEquals;
import static org.mockito.Mockito.mock;
import android.os.Build;
import android.util.Range;
import io.flutter.plugins.camera.CameraProperties;
import io.flutter.plugins.camera.utils.TestUtils;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.robolectric.RobolectricTestRunner;
@RunWith(RobolectricTestRunner.class)
public class FpsRangeFeaturePixel4aTest {
@Test
public void ctor_shouldInitializeFpsRangeWith30WhenDeviceIsPixel4a() {
TestUtils.setFinalStatic(Build.class, "BRAND", "google");
TestUtils.setFinalStatic(Build.class, "MODEL", "Pixel 4a");
FpsRangeFeature fpsRangeFeature = new FpsRangeFeature(mock(CameraProperties.class));
Range<Integer> range = fpsRangeFeature.getValue();
assertEquals(30, (int) range.getLower());
assertEquals(30, (int) range.getUpper());
}
}
| plugins/packages/camera/camera_android/android/src/test/java/io/flutter/plugins/camera/features/fpsrange/FpsRangeFeaturePixel4aTest.java/0 | {
"file_path": "plugins/packages/camera/camera_android/android/src/test/java/io/flutter/plugins/camera/features/fpsrange/FpsRangeFeaturePixel4aTest.java",
"repo_id": "plugins",
"token_count": 364
} | 1,190 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart' show BinaryMessenger;
import 'android_camera_camerax_flutter_api_impls.dart';
import 'camerax_library.g.dart';
import 'instance_manager.dart';
import 'java_object.dart';
/// The interface used to control the flow of data of use cases, control the
/// camera, and publich the state of the camera.
///
/// See https://developer.android.com/reference/androidx/camera/core/Camera.
class Camera extends JavaObject {
/// Constructs a [Camera] that is not automatically attached to a native object.
Camera.detached({super.binaryMessenger, super.instanceManager})
: super.detached() {
AndroidCameraXCameraFlutterApis.instance.ensureSetUp();
}
}
/// Flutter API implementation of [Camera].
class CameraFlutterApiImpl implements CameraFlutterApi {
/// Constructs a [CameraSelectorFlutterApiImpl].
CameraFlutterApiImpl({
this.binaryMessenger,
InstanceManager? instanceManager,
}) : instanceManager = instanceManager ?? JavaObject.globalInstanceManager;
/// Receives binary data across the Flutter platform barrier.
///
/// If it is null, the default BinaryMessenger will be used which routes to
/// the host platform.
final BinaryMessenger? binaryMessenger;
/// Maintains instances stored to communicate with native language objects.
final InstanceManager instanceManager;
@override
void create(int identifier) {
instanceManager.addHostCreatedInstance(
Camera.detached(
binaryMessenger: binaryMessenger, instanceManager: instanceManager),
identifier,
onCopy: (Camera original) {
return Camera.detached(
binaryMessenger: binaryMessenger, instanceManager: instanceManager);
},
);
}
}
| plugins/packages/camera/camera_android_camerax/lib/src/camera.dart/0 | {
"file_path": "plugins/packages/camera/camera_android_camerax/lib/src/camera.dart",
"repo_id": "plugins",
"token_count": 562
} | 1,191 |
// Mocks generated by Mockito 5.3.2 from annotations
// in camera_android_camerax/test/camera_info_test.dart.
// Do not manually edit this file.
// ignore_for_file: no_leading_underscores_for_library_prefixes
import 'package:mockito/mockito.dart' as _i1;
import 'test_camerax_library.g.dart' as _i2;
// ignore_for_file: type=lint
// ignore_for_file: avoid_redundant_argument_values
// ignore_for_file: avoid_setters_without_getters
// ignore_for_file: comment_references
// ignore_for_file: implementation_imports
// ignore_for_file: invalid_use_of_visible_for_testing_member
// ignore_for_file: prefer_const_constructors
// ignore_for_file: unnecessary_parenthesis
// ignore_for_file: camel_case_types
// ignore_for_file: subtype_of_sealed_class
/// A class which mocks [TestCameraInfoHostApi].
///
/// See the documentation for Mockito's code generation for more information.
class MockTestCameraInfoHostApi extends _i1.Mock
implements _i2.TestCameraInfoHostApi {
MockTestCameraInfoHostApi() {
_i1.throwOnMissingStub(this);
}
@override
int getSensorRotationDegrees(int? identifier) => (super.noSuchMethod(
Invocation.method(
#getSensorRotationDegrees,
[identifier],
),
returnValue: 0,
) as int);
}
| plugins/packages/camera/camera_android_camerax/test/camera_info_test.mocks.dart/0 | {
"file_path": "plugins/packages/camera/camera_android_camerax/test/camera_info_test.mocks.dart",
"repo_id": "plugins",
"token_count": 463
} | 1,192 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:io';
import 'dart:ui';
import 'package:camera_avfoundation/camera_avfoundation.dart';
import 'package:camera_example/camera_controller.dart';
import 'package:camera_platform_interface/camera_platform_interface.dart';
import 'package:flutter/painting.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:integration_test/integration_test.dart';
import 'package:path_provider/path_provider.dart';
import 'package:video_player/video_player.dart';
void main() {
late Directory testDir;
IntegrationTestWidgetsFlutterBinding.ensureInitialized();
setUpAll(() async {
CameraPlatform.instance = AVFoundationCamera();
final Directory extDir = await getTemporaryDirectory();
testDir = await Directory('${extDir.path}/test').create(recursive: true);
});
tearDownAll(() async {
await testDir.delete(recursive: true);
});
final Map<ResolutionPreset, Size> presetExpectedSizes =
<ResolutionPreset, Size>{
ResolutionPreset.low: const Size(288, 352),
ResolutionPreset.medium: const Size(480, 640),
ResolutionPreset.high: const Size(720, 1280),
ResolutionPreset.veryHigh: const Size(1080, 1920),
ResolutionPreset.ultraHigh: const Size(2160, 3840),
// Don't bother checking for max here since it could be anything.
};
/// Verify that [actual] has dimensions that are at least as large as
/// [expectedSize]. Allows for a mismatch in portrait vs landscape. Returns
/// whether the dimensions exactly match.
bool assertExpectedDimensions(Size expectedSize, Size actual) {
expect(actual.shortestSide, lessThanOrEqualTo(expectedSize.shortestSide));
expect(actual.longestSide, lessThanOrEqualTo(expectedSize.longestSide));
return actual.shortestSide == expectedSize.shortestSide &&
actual.longestSide == expectedSize.longestSide;
}
// This tests that the capture is no bigger than the preset, since we have
// automatic code to fall back to smaller sizes when we need to. Returns
// whether the image is exactly the desired resolution.
Future<bool> testCaptureImageResolution(
CameraController controller, ResolutionPreset preset) async {
final Size expectedSize = presetExpectedSizes[preset]!;
// Take Picture
final XFile file = await controller.takePicture();
// Load picture
final File fileImage = File(file.path);
final Image image = await decodeImageFromList(fileImage.readAsBytesSync());
// Verify image dimensions are as expected
expect(image, isNotNull);
return assertExpectedDimensions(
expectedSize, Size(image.height.toDouble(), image.width.toDouble()));
}
testWidgets('Capture specific image resolutions',
(WidgetTester tester) async {
final List<CameraDescription> cameras =
await CameraPlatform.instance.availableCameras();
if (cameras.isEmpty) {
return;
}
for (final CameraDescription cameraDescription in cameras) {
bool previousPresetExactlySupported = true;
for (final MapEntry<ResolutionPreset, Size> preset
in presetExpectedSizes.entries) {
final CameraController controller =
CameraController(cameraDescription, preset.key);
await controller.initialize();
final bool presetExactlySupported =
await testCaptureImageResolution(controller, preset.key);
assert(!(!previousPresetExactlySupported && presetExactlySupported),
'The camera took higher resolution pictures at a lower resolution.');
previousPresetExactlySupported = presetExactlySupported;
await controller.dispose();
}
}
});
// This tests that the capture is no bigger than the preset, since we have
// automatic code to fall back to smaller sizes when we need to. Returns
// whether the image is exactly the desired resolution.
Future<bool> testCaptureVideoResolution(
CameraController controller, ResolutionPreset preset) async {
final Size expectedSize = presetExpectedSizes[preset]!;
// Take Video
await controller.startVideoRecording();
sleep(const Duration(milliseconds: 300));
final XFile file = await controller.stopVideoRecording();
// Load video metadata
final File videoFile = File(file.path);
final VideoPlayerController videoController =
VideoPlayerController.file(videoFile);
await videoController.initialize();
final Size video = videoController.value.size;
// Verify image dimensions are as expected
expect(video, isNotNull);
return assertExpectedDimensions(
expectedSize, Size(video.height, video.width));
}
testWidgets('Capture specific video resolutions',
(WidgetTester tester) async {
final List<CameraDescription> cameras =
await CameraPlatform.instance.availableCameras();
if (cameras.isEmpty) {
return;
}
for (final CameraDescription cameraDescription in cameras) {
bool previousPresetExactlySupported = true;
for (final MapEntry<ResolutionPreset, Size> preset
in presetExpectedSizes.entries) {
final CameraController controller =
CameraController(cameraDescription, preset.key);
await controller.initialize();
await controller.prepareForVideoRecording();
final bool presetExactlySupported =
await testCaptureVideoResolution(controller, preset.key);
assert(!(!previousPresetExactlySupported && presetExactlySupported),
'The camera took higher resolution pictures at a lower resolution.');
previousPresetExactlySupported = presetExactlySupported;
await controller.dispose();
}
}
});
testWidgets('Pause and resume video recording', (WidgetTester tester) async {
final List<CameraDescription> cameras =
await CameraPlatform.instance.availableCameras();
if (cameras.isEmpty) {
return;
}
final CameraController controller = CameraController(
cameras[0],
ResolutionPreset.low,
enableAudio: false,
);
await controller.initialize();
await controller.prepareForVideoRecording();
int startPause;
int timePaused = 0;
await controller.startVideoRecording();
final int recordingStart = DateTime.now().millisecondsSinceEpoch;
sleep(const Duration(milliseconds: 500));
await controller.pauseVideoRecording();
startPause = DateTime.now().millisecondsSinceEpoch;
sleep(const Duration(milliseconds: 500));
await controller.resumeVideoRecording();
timePaused += DateTime.now().millisecondsSinceEpoch - startPause;
sleep(const Duration(milliseconds: 500));
await controller.pauseVideoRecording();
startPause = DateTime.now().millisecondsSinceEpoch;
sleep(const Duration(milliseconds: 500));
await controller.resumeVideoRecording();
timePaused += DateTime.now().millisecondsSinceEpoch - startPause;
sleep(const Duration(milliseconds: 500));
final XFile file = await controller.stopVideoRecording();
final int recordingTime =
DateTime.now().millisecondsSinceEpoch - recordingStart;
final File videoFile = File(file.path);
final VideoPlayerController videoController = VideoPlayerController.file(
videoFile,
);
await videoController.initialize();
final int duration = videoController.value.duration.inMilliseconds;
await videoController.dispose();
expect(duration, lessThan(recordingTime - timePaused));
});
/// Start streaming with specifying the ImageFormatGroup.
Future<CameraImageData> startStreaming(List<CameraDescription> cameras,
ImageFormatGroup? imageFormatGroup) async {
final CameraController controller = CameraController(
cameras.first,
ResolutionPreset.low,
enableAudio: false,
imageFormatGroup: imageFormatGroup,
);
await controller.initialize();
final Completer<CameraImageData> completer = Completer<CameraImageData>();
await controller.startImageStream((CameraImageData image) {
if (!completer.isCompleted) {
Future<void>(() async {
await controller.stopImageStream();
await controller.dispose();
}).then((Object? value) {
completer.complete(image);
});
}
});
return completer.future;
}
testWidgets(
'image streaming with imageFormatGroup',
(WidgetTester tester) async {
final List<CameraDescription> cameras =
await CameraPlatform.instance.availableCameras();
if (cameras.isEmpty) {
return;
}
CameraImageData image = await startStreaming(cameras, null);
expect(image, isNotNull);
expect(image.format.group, ImageFormatGroup.bgra8888);
expect(image.planes.length, 1);
image = await startStreaming(cameras, ImageFormatGroup.yuv420);
expect(image, isNotNull);
expect(image.format.group, ImageFormatGroup.yuv420);
expect(image.planes.length, 2);
image = await startStreaming(cameras, ImageFormatGroup.bgra8888);
expect(image, isNotNull);
expect(image.format.group, ImageFormatGroup.bgra8888);
expect(image.planes.length, 1);
},
);
testWidgets('Recording with video streaming', (WidgetTester tester) async {
final List<CameraDescription> cameras =
await CameraPlatform.instance.availableCameras();
if (cameras.isEmpty) {
return;
}
final CameraController controller = CameraController(
cameras[0],
ResolutionPreset.low,
enableAudio: false,
);
await controller.initialize();
await controller.prepareForVideoRecording();
final Completer<CameraImageData> completer = Completer<CameraImageData>();
await controller.startVideoRecording(
streamCallback: (CameraImageData image) {
if (!completer.isCompleted) {
completer.complete(image);
}
});
sleep(const Duration(milliseconds: 500));
await controller.stopVideoRecording();
await controller.dispose();
expect(await completer.future, isNotNull);
});
}
| plugins/packages/camera/camera_avfoundation/example/integration_test/camera_test.dart/0 | {
"file_path": "plugins/packages/camera/camera_avfoundation/example/integration_test/camera_test.dart",
"repo_id": "plugins",
"token_count": 3356
} | 1,193 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
@import camera_avfoundation;
@import camera_avfoundation.Test;
@import AVFoundation;
@import XCTest;
#import <OCMock/OCMock.h>
#import "CameraTestUtils.h"
/// Includes test cases related to photo capture operations for FLTCam class.
@interface FLTCamPhotoCaptureTests : XCTestCase
@end
@implementation FLTCamPhotoCaptureTests
- (void)testCaptureToFile_mustReportErrorToResultIfSavePhotoDelegateCompletionsWithError {
XCTestExpectation *errorExpectation =
[self expectationWithDescription:
@"Must send error to result if save photo delegate completes with error."];
dispatch_queue_t captureSessionQueue = dispatch_queue_create("capture_session_queue", NULL);
dispatch_queue_set_specific(captureSessionQueue, FLTCaptureSessionQueueSpecific,
(void *)FLTCaptureSessionQueueSpecific, NULL);
FLTCam *cam = FLTCreateCamWithCaptureSessionQueue(captureSessionQueue);
AVCapturePhotoSettings *settings = [AVCapturePhotoSettings photoSettings];
id mockSettings = OCMClassMock([AVCapturePhotoSettings class]);
OCMStub([mockSettings photoSettings]).andReturn(settings);
NSError *error = [NSError errorWithDomain:@"test" code:0 userInfo:nil];
id mockResult = OCMClassMock([FLTThreadSafeFlutterResult class]);
OCMStub([mockResult sendError:error]).andDo(^(NSInvocation *invocation) {
[errorExpectation fulfill];
});
id mockOutput = OCMClassMock([AVCapturePhotoOutput class]);
OCMStub([mockOutput capturePhotoWithSettings:OCMOCK_ANY delegate:OCMOCK_ANY])
.andDo(^(NSInvocation *invocation) {
FLTSavePhotoDelegate *delegate = cam.inProgressSavePhotoDelegates[@(settings.uniqueID)];
// Completion runs on IO queue.
dispatch_queue_t ioQueue = dispatch_queue_create("io_queue", NULL);
dispatch_async(ioQueue, ^{
delegate.completionHandler(nil, error);
});
});
cam.capturePhotoOutput = mockOutput;
// `FLTCam::captureToFile` runs on capture session queue.
dispatch_async(captureSessionQueue, ^{
[cam captureToFile:mockResult];
});
[self waitForExpectationsWithTimeout:1 handler:nil];
}
- (void)testCaptureToFile_mustReportPathToResultIfSavePhotoDelegateCompletionsWithPath {
XCTestExpectation *pathExpectation =
[self expectationWithDescription:
@"Must send file path to result if save photo delegate completes with file path."];
dispatch_queue_t captureSessionQueue = dispatch_queue_create("capture_session_queue", NULL);
dispatch_queue_set_specific(captureSessionQueue, FLTCaptureSessionQueueSpecific,
(void *)FLTCaptureSessionQueueSpecific, NULL);
FLTCam *cam = FLTCreateCamWithCaptureSessionQueue(captureSessionQueue);
AVCapturePhotoSettings *settings = [AVCapturePhotoSettings photoSettings];
id mockSettings = OCMClassMock([AVCapturePhotoSettings class]);
OCMStub([mockSettings photoSettings]).andReturn(settings);
NSString *filePath = @"test";
id mockResult = OCMClassMock([FLTThreadSafeFlutterResult class]);
OCMStub([mockResult sendSuccessWithData:filePath]).andDo(^(NSInvocation *invocation) {
[pathExpectation fulfill];
});
id mockOutput = OCMClassMock([AVCapturePhotoOutput class]);
OCMStub([mockOutput capturePhotoWithSettings:OCMOCK_ANY delegate:OCMOCK_ANY])
.andDo(^(NSInvocation *invocation) {
FLTSavePhotoDelegate *delegate = cam.inProgressSavePhotoDelegates[@(settings.uniqueID)];
// Completion runs on IO queue.
dispatch_queue_t ioQueue = dispatch_queue_create("io_queue", NULL);
dispatch_async(ioQueue, ^{
delegate.completionHandler(filePath, nil);
});
});
cam.capturePhotoOutput = mockOutput;
// `FLTCam::captureToFile` runs on capture session queue.
dispatch_async(captureSessionQueue, ^{
[cam captureToFile:mockResult];
});
[self waitForExpectationsWithTimeout:1 handler:nil];
}
@end
| plugins/packages/camera/camera_avfoundation/example/ios/RunnerTests/FLTCamPhotoCaptureTests.m/0 | {
"file_path": "plugins/packages/camera/camera_avfoundation/example/ios/RunnerTests/FLTCamPhotoCaptureTests.m",
"repo_id": "plugins",
"token_count": 1391
} | 1,194 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#import <Flutter/Flutter.h>
NS_ASSUME_NONNULL_BEGIN
/**
* A thread safe wrapper for FlutterEventChannel that can be called from any thread, by dispatching
* its underlying engine calls to the main thread.
*/
@interface FLTThreadSafeEventChannel : NSObject
/**
* Creates a FLTThreadSafeEventChannel by wrapping a FlutterEventChannel object.
* @param channel The FlutterEventChannel object to be wrapped.
*/
- (instancetype)initWithEventChannel:(FlutterEventChannel *)channel;
/*
* Registers a handler on the main thread for stream setup requests from the Flutter side.
# The completion block runs on the main thread.
*/
- (void)setStreamHandler:(nullable NSObject<FlutterStreamHandler> *)handler
completion:(void (^)(void))completion;
@end
NS_ASSUME_NONNULL_END
| plugins/packages/camera/camera_avfoundation/ios/Classes/FLTThreadSafeEventChannel.h/0 | {
"file_path": "plugins/packages/camera/camera_avfoundation/ios/Classes/FLTThreadSafeEventChannel.h",
"repo_id": "plugins",
"token_count": 275
} | 1,195 |
name: camera_avfoundation
description: iOS implementation of the camera plugin.
repository: https://github.com/flutter/plugins/tree/main/packages/camera/camera_avfoundation
issue_tracker: https://github.com/flutter/flutter/issues?q=is%3Aissue+is%3Aopen+label%3A%22p%3A+camera%22
version: 0.9.11
environment:
sdk: ">=2.14.0 <3.0.0"
flutter: ">=3.0.0"
flutter:
plugin:
implements: camera
platforms:
ios:
pluginClass: CameraPlugin
dartPluginClass: AVFoundationCamera
dependencies:
camera_platform_interface: ^2.3.1
flutter:
sdk: flutter
stream_transform: ^2.0.0
dev_dependencies:
async: ^2.5.0
flutter_driver:
sdk: flutter
flutter_test:
sdk: flutter
| plugins/packages/camera/camera_avfoundation/pubspec.yaml/0 | {
"file_path": "plugins/packages/camera/camera_avfoundation/pubspec.yaml",
"repo_id": "plugins",
"token_count": 293
} | 1,196 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
/// This is thrown when the plugin reports an error.
class CameraException implements Exception {
/// Creates a new camera exception with the given error code and description.
CameraException(this.code, this.description);
/// Error code.
// TODO(bparrishMines): Document possible error codes.
// https://github.com/flutter/flutter/issues/69298
String code;
/// Textual description of the error.
String? description;
@override
String toString() => 'CameraException($code, $description)';
}
| plugins/packages/camera/camera_platform_interface/lib/src/types/camera_exception.dart/0 | {
"file_path": "plugins/packages/camera/camera_platform_interface/lib/src/types/camera_exception.dart",
"repo_id": "plugins",
"token_count": 178
} | 1,197 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:camera_platform_interface/camera_platform_interface.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
group('CameraLensDirection tests', () {
test('CameraLensDirection should contain 3 options', () {
const List<CameraLensDirection> values = CameraLensDirection.values;
expect(values.length, 3);
});
test('CameraLensDirection enum should have items in correct index', () {
const List<CameraLensDirection> values = CameraLensDirection.values;
expect(values[0], CameraLensDirection.front);
expect(values[1], CameraLensDirection.back);
expect(values[2], CameraLensDirection.external);
});
});
group('CameraDescription tests', () {
test('Constructor should initialize all properties', () {
const CameraDescription description = CameraDescription(
name: 'Test',
lensDirection: CameraLensDirection.front,
sensorOrientation: 90,
);
expect(description.name, 'Test');
expect(description.lensDirection, CameraLensDirection.front);
expect(description.sensorOrientation, 90);
});
test('equals should return true if objects are the same', () {
const CameraDescription firstDescription = CameraDescription(
name: 'Test',
lensDirection: CameraLensDirection.front,
sensorOrientation: 90,
);
const CameraDescription secondDescription = CameraDescription(
name: 'Test',
lensDirection: CameraLensDirection.front,
sensorOrientation: 90,
);
expect(firstDescription == secondDescription, true);
});
test('equals should return false if name is different', () {
const CameraDescription firstDescription = CameraDescription(
name: 'Test',
lensDirection: CameraLensDirection.front,
sensorOrientation: 90,
);
const CameraDescription secondDescription = CameraDescription(
name: 'Testing',
lensDirection: CameraLensDirection.front,
sensorOrientation: 90,
);
expect(firstDescription == secondDescription, false);
});
test('equals should return false if lens direction is different', () {
const CameraDescription firstDescription = CameraDescription(
name: 'Test',
lensDirection: CameraLensDirection.front,
sensorOrientation: 90,
);
const CameraDescription secondDescription = CameraDescription(
name: 'Test',
lensDirection: CameraLensDirection.back,
sensorOrientation: 90,
);
expect(firstDescription == secondDescription, false);
});
test('equals should return true if sensor orientation is different', () {
const CameraDescription firstDescription = CameraDescription(
name: 'Test',
lensDirection: CameraLensDirection.front,
sensorOrientation: 0,
);
const CameraDescription secondDescription = CameraDescription(
name: 'Test',
lensDirection: CameraLensDirection.front,
sensorOrientation: 90,
);
expect(firstDescription == secondDescription, true);
});
test('hashCode should match hashCode of all equality-tested properties',
() {
const CameraDescription description = CameraDescription(
name: 'Test',
lensDirection: CameraLensDirection.front,
sensorOrientation: 0,
);
final int expectedHashCode =
Object.hash(description.name, description.lensDirection);
expect(description.hashCode, expectedHashCode);
});
});
}
| plugins/packages/camera/camera_platform_interface/test/types/camera_description_test.dart/0 | {
"file_path": "plugins/packages/camera/camera_platform_interface/test/types/camera_description_test.dart",
"repo_id": "plugins",
"token_count": 1305
} | 1,198 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:html' as html;
// TODO(a14n): remove this import once Flutter 3.1 or later reaches stable (including flutter/flutter#106316)
// ignore: unnecessary_import
import 'dart:ui';
import 'package:camera_platform_interface/camera_platform_interface.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/services.dart';
import 'camera.dart';
import 'shims/dart_js_util.dart';
import 'types/types.dart';
/// A service to fetch, map camera settings and
/// obtain the camera stream.
class CameraService {
// A facing mode constraint name.
static const String _facingModeKey = 'facingMode';
/// The current browser window used to access media devices.
@visibleForTesting
html.Window? window = html.window;
/// The utility to manipulate JavaScript interop objects.
@visibleForTesting
JsUtil jsUtil = JsUtil();
/// Returns a media stream associated with the camera device
/// with [cameraId] and constrained by [options].
Future<html.MediaStream> getMediaStreamForOptions(
CameraOptions options, {
int cameraId = 0,
}) async {
final html.MediaDevices? mediaDevices = window?.navigator.mediaDevices;
// Throw a not supported exception if the current browser window
// does not support any media devices.
if (mediaDevices == null) {
throw PlatformException(
code: CameraErrorCode.notSupported.toString(),
message: 'The camera is not supported on this device.',
);
}
try {
final Map<String, dynamic> constraints = options.toJson();
return await mediaDevices.getUserMedia(constraints);
} on html.DomException catch (e) {
switch (e.name) {
case 'NotFoundError':
case 'DevicesNotFoundError':
throw CameraWebException(
cameraId,
CameraErrorCode.notFound,
'No camera found for the given camera options.',
);
case 'NotReadableError':
case 'TrackStartError':
throw CameraWebException(
cameraId,
CameraErrorCode.notReadable,
'The camera is not readable due to a hardware error '
'that prevented access to the device.',
);
case 'OverconstrainedError':
case 'ConstraintNotSatisfiedError':
throw CameraWebException(
cameraId,
CameraErrorCode.overconstrained,
'The camera options are impossible to satisfy.',
);
case 'NotAllowedError':
case 'PermissionDeniedError':
throw CameraWebException(
cameraId,
CameraErrorCode.permissionDenied,
'The camera cannot be used or the permission '
'to access the camera is not granted.',
);
case 'TypeError':
throw CameraWebException(
cameraId,
CameraErrorCode.type,
'The camera options are incorrect or attempted '
'to access the media input from an insecure context.',
);
case 'AbortError':
throw CameraWebException(
cameraId,
CameraErrorCode.abort,
'Some problem occurred that prevented the camera from being used.',
);
case 'SecurityError':
throw CameraWebException(
cameraId,
CameraErrorCode.security,
'The user media support is disabled in the current browser.',
);
default:
throw CameraWebException(
cameraId,
CameraErrorCode.unknown,
'An unknown error occured when fetching the camera stream.',
);
}
} catch (_) {
throw CameraWebException(
cameraId,
CameraErrorCode.unknown,
'An unknown error occured when fetching the camera stream.',
);
}
}
/// Returns the zoom level capability for the given [camera].
///
/// Throws a [CameraWebException] if the zoom level is not supported
/// or the camera has not been initialized or started.
ZoomLevelCapability getZoomLevelCapabilityForCamera(
Camera camera,
) {
final html.MediaDevices? mediaDevices = window?.navigator.mediaDevices;
final Map<dynamic, dynamic>? supportedConstraints =
mediaDevices?.getSupportedConstraints();
final bool zoomLevelSupported =
supportedConstraints?[ZoomLevelCapability.constraintName] as bool? ??
false;
if (!zoomLevelSupported) {
throw CameraWebException(
camera.textureId,
CameraErrorCode.zoomLevelNotSupported,
'The zoom level is not supported in the current browser.',
);
}
final List<html.MediaStreamTrack> videoTracks =
camera.stream?.getVideoTracks() ?? <html.MediaStreamTrack>[];
if (videoTracks.isNotEmpty) {
final html.MediaStreamTrack defaultVideoTrack = videoTracks.first;
/// The zoom level capability is represented by MediaSettingsRange.
/// See: https://developer.mozilla.org/en-US/docs/Web/API/MediaSettingsRange
final Object zoomLevelCapability = defaultVideoTrack
.getCapabilities()[ZoomLevelCapability.constraintName]
as Object? ??
<dynamic, dynamic>{};
// The zoom level capability is a nested JS object, therefore
// we need to access its properties with the js_util library.
// See: https://api.dart.dev/stable/2.13.4/dart-js_util/getProperty.html
final num? minimumZoomLevel =
jsUtil.getProperty(zoomLevelCapability, 'min') as num?;
final num? maximumZoomLevel =
jsUtil.getProperty(zoomLevelCapability, 'max') as num?;
if (minimumZoomLevel != null && maximumZoomLevel != null) {
return ZoomLevelCapability(
minimum: minimumZoomLevel.toDouble(),
maximum: maximumZoomLevel.toDouble(),
videoTrack: defaultVideoTrack,
);
} else {
throw CameraWebException(
camera.textureId,
CameraErrorCode.zoomLevelNotSupported,
'The zoom level is not supported by the current camera.',
);
}
} else {
throw CameraWebException(
camera.textureId,
CameraErrorCode.notStarted,
'The camera has not been initialized or started.',
);
}
}
/// Returns a facing mode of the [videoTrack]
/// (null if the facing mode is not available).
String? getFacingModeForVideoTrack(html.MediaStreamTrack videoTrack) {
final html.MediaDevices? mediaDevices = window?.navigator.mediaDevices;
// Throw a not supported exception if the current browser window
// does not support any media devices.
if (mediaDevices == null) {
throw PlatformException(
code: CameraErrorCode.notSupported.toString(),
message: 'The camera is not supported on this device.',
);
}
// Check if the camera facing mode is supported by the current browser.
final Map<dynamic, dynamic> supportedConstraints =
mediaDevices.getSupportedConstraints();
final bool facingModeSupported =
supportedConstraints[_facingModeKey] as bool? ?? false;
// Return null if the facing mode is not supported.
if (!facingModeSupported) {
return null;
}
// Extract the facing mode from the video track settings.
// The property may not be available if it's not supported
// by the browser or not available due to context.
//
// MediaTrackSettings:
// https://developer.mozilla.org/en-US/docs/Web/API/MediaTrackSettings
final Map<dynamic, dynamic> videoTrackSettings = videoTrack.getSettings();
final String? facingMode = videoTrackSettings[_facingModeKey] as String?;
if (facingMode == null) {
// If the facing mode does not exist in the video track settings,
// check for the facing mode in the video track capabilities.
//
// MediaTrackCapabilities:
// https://www.w3.org/TR/mediacapture-streams/#dom-mediatrackcapabilities
// Check if getting the video track capabilities is supported.
//
// The method may not be supported on Firefox.
// See: https://developer.mozilla.org/en-US/docs/Web/API/MediaStreamTrack/getCapabilities#browser_compatibility
if (!jsUtil.hasProperty(videoTrack, 'getCapabilities')) {
// Return null if the video track capabilites are not supported.
return null;
}
final Map<dynamic, dynamic> videoTrackCapabilities =
videoTrack.getCapabilities();
// A list of facing mode capabilities as
// the camera may support multiple facing modes.
final List<String> facingModeCapabilities = List<String>.from(
(videoTrackCapabilities[_facingModeKey] as List<dynamic>?)
?.cast<String>() ??
<String>[]);
if (facingModeCapabilities.isNotEmpty) {
final String facingModeCapability = facingModeCapabilities.first;
return facingModeCapability;
} else {
// Return null if there are no facing mode capabilities.
return null;
}
}
return facingMode;
}
/// Maps the given [facingMode] to [CameraLensDirection].
///
/// The following values for the facing mode are supported:
/// https://developer.mozilla.org/en-US/docs/Web/API/MediaTrackSettings/facingMode
CameraLensDirection mapFacingModeToLensDirection(String facingMode) {
switch (facingMode) {
case 'user':
return CameraLensDirection.front;
case 'environment':
return CameraLensDirection.back;
case 'left':
case 'right':
default:
return CameraLensDirection.external;
}
}
/// Maps the given [facingMode] to [CameraType].
///
/// See [CameraMetadata.facingMode] for more details.
CameraType mapFacingModeToCameraType(String facingMode) {
switch (facingMode) {
case 'user':
return CameraType.user;
case 'environment':
return CameraType.environment;
case 'left':
case 'right':
default:
return CameraType.user;
}
}
/// Maps the given [resolutionPreset] to [Size].
Size mapResolutionPresetToSize(ResolutionPreset resolutionPreset) {
switch (resolutionPreset) {
case ResolutionPreset.max:
case ResolutionPreset.ultraHigh:
return const Size(4096, 2160);
case ResolutionPreset.veryHigh:
return const Size(1920, 1080);
case ResolutionPreset.high:
return const Size(1280, 720);
case ResolutionPreset.medium:
return const Size(720, 480);
case ResolutionPreset.low:
return const Size(320, 240);
}
// The enum comes from a different package, which could get a new value at
// any time, so provide a fallback that ensures this won't break when used
// with a version that contains new values. This is deliberately outside
// the switch rather than a `default` so that the linter will flag the
// switch as needing an update.
// ignore: dead_code
return const Size(320, 240);
}
/// Maps the given [deviceOrientation] to [OrientationType].
String mapDeviceOrientationToOrientationType(
DeviceOrientation deviceOrientation,
) {
switch (deviceOrientation) {
case DeviceOrientation.portraitUp:
return OrientationType.portraitPrimary;
case DeviceOrientation.landscapeLeft:
return OrientationType.landscapePrimary;
case DeviceOrientation.portraitDown:
return OrientationType.portraitSecondary;
case DeviceOrientation.landscapeRight:
return OrientationType.landscapeSecondary;
}
}
/// Maps the given [orientationType] to [DeviceOrientation].
DeviceOrientation mapOrientationTypeToDeviceOrientation(
String orientationType,
) {
switch (orientationType) {
case OrientationType.portraitPrimary:
return DeviceOrientation.portraitUp;
case OrientationType.landscapePrimary:
return DeviceOrientation.landscapeLeft;
case OrientationType.portraitSecondary:
return DeviceOrientation.portraitDown;
case OrientationType.landscapeSecondary:
return DeviceOrientation.landscapeRight;
default:
return DeviceOrientation.portraitUp;
}
}
}
| plugins/packages/camera/camera_web/lib/src/camera_service.dart/0 | {
"file_path": "plugins/packages/camera/camera_web/lib/src/camera_service.dart",
"repo_id": "plugins",
"token_count": 4574
} | 1,199 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// ignore_for_file: avoid_print
import 'package:flutter_test/flutter_test.dart';
void main() {
test('Tell the user where to find more tests', () {
print('---');
print('This package also uses integration_test for its tests.');
print('See `example/README.md` for more info.');
print('---');
});
}
| plugins/packages/camera/camera_web/test/more_tests_exist_elsewhere_test.dart/0 | {
"file_path": "plugins/packages/camera/camera_web/test/more_tests_exist_elsewhere_test.dart",
"repo_id": "plugins",
"token_count": 152
} | 1,200 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:async/async.dart';
import 'package:camera_platform_interface/camera_platform_interface.dart';
import 'package:camera_windows/camera_windows.dart';
import 'package:flutter/services.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
import './utils/method_channel_mock.dart';
void main() {
const String pluginChannelName = 'plugins.flutter.io/camera_windows';
TestWidgetsFlutterBinding.ensureInitialized();
group('$CameraWindows()', () {
test('registered instance', () {
CameraWindows.registerWith();
expect(CameraPlatform.instance, isA<CameraWindows>());
});
group('Creation, Initialization & Disposal Tests', () {
test('Should send creation data and receive back a camera id', () async {
// Arrange
final MethodChannelMock cameraMockChannel = MethodChannelMock(
channelName: pluginChannelName,
methods: <String, dynamic>{
'create': <String, dynamic>{
'cameraId': 1,
'imageFormatGroup': 'unknown',
}
});
final CameraWindows plugin = CameraWindows();
// Act
final int cameraId = await plugin.createCamera(
const CameraDescription(
name: 'Test',
lensDirection: CameraLensDirection.front,
sensorOrientation: 0),
ResolutionPreset.high,
);
// Assert
expect(cameraMockChannel.log, <Matcher>[
isMethodCall(
'create',
arguments: <String, Object?>{
'cameraName': 'Test',
'resolutionPreset': 'high',
'enableAudio': false
},
),
]);
expect(cameraId, 1);
});
test(
'Should throw CameraException when create throws a PlatformException',
() {
// Arrange
MethodChannelMock(
channelName: pluginChannelName,
methods: <String, dynamic>{
'create': PlatformException(
code: 'TESTING_ERROR_CODE',
message: 'Mock error message used during testing.',
)
});
final CameraWindows plugin = CameraWindows();
// Act
expect(
() => plugin.createCamera(
const CameraDescription(
name: 'Test',
lensDirection: CameraLensDirection.back,
sensorOrientation: 0,
),
ResolutionPreset.high,
),
throwsA(
isA<CameraException>()
.having(
(CameraException e) => e.code, 'code', 'TESTING_ERROR_CODE')
.having((CameraException e) => e.description, 'description',
'Mock error message used during testing.'),
),
);
});
test(
'Should throw CameraException when initialize throws a PlatformException',
() {
// Arrange
MethodChannelMock(
channelName: pluginChannelName,
methods: <String, dynamic>{
'initialize': PlatformException(
code: 'TESTING_ERROR_CODE',
message: 'Mock error message used during testing.',
)
},
);
final CameraWindows plugin = CameraWindows();
// Act
expect(
() => plugin.initializeCamera(0),
throwsA(
isA<CameraException>()
.having((CameraException e) => e.code, 'code',
'TESTING_ERROR_CODE')
.having(
(CameraException e) => e.description,
'description',
'Mock error message used during testing.',
),
),
);
},
);
test('Should send initialization data', () async {
// Arrange
final MethodChannelMock cameraMockChannel = MethodChannelMock(
channelName: pluginChannelName,
methods: <String, dynamic>{
'create': <String, dynamic>{
'cameraId': 1,
'imageFormatGroup': 'unknown',
},
'initialize': <String, dynamic>{
'previewWidth': 1920.toDouble(),
'previewHeight': 1080.toDouble()
},
});
final CameraWindows plugin = CameraWindows();
final int cameraId = await plugin.createCamera(
const CameraDescription(
name: 'Test',
lensDirection: CameraLensDirection.back,
sensorOrientation: 0,
),
ResolutionPreset.high,
);
// Act
await plugin.initializeCamera(cameraId);
// Assert
expect(cameraId, 1);
expect(cameraMockChannel.log, <Matcher>[
anything,
isMethodCall(
'initialize',
arguments: <String, Object?>{'cameraId': 1},
),
]);
});
test('Should send a disposal call on dispose', () async {
// Arrange
final MethodChannelMock cameraMockChannel = MethodChannelMock(
channelName: pluginChannelName,
methods: <String, dynamic>{
'create': <String, dynamic>{'cameraId': 1},
'initialize': <String, dynamic>{
'previewWidth': 1920.toDouble(),
'previewHeight': 1080.toDouble()
},
'dispose': <String, dynamic>{'cameraId': 1}
});
final CameraWindows plugin = CameraWindows();
final int cameraId = await plugin.createCamera(
const CameraDescription(
name: 'Test',
lensDirection: CameraLensDirection.back,
sensorOrientation: 0,
),
ResolutionPreset.high,
);
await plugin.initializeCamera(cameraId);
// Act
await plugin.dispose(cameraId);
// Assert
expect(cameraId, 1);
expect(cameraMockChannel.log, <Matcher>[
anything,
anything,
isMethodCall(
'dispose',
arguments: <String, Object?>{'cameraId': 1},
),
]);
});
});
group('Event Tests', () {
late CameraWindows plugin;
late int cameraId;
setUp(() async {
MethodChannelMock(
channelName: pluginChannelName,
methods: <String, dynamic>{
'create': <String, dynamic>{'cameraId': 1},
'initialize': <String, dynamic>{
'previewWidth': 1920.toDouble(),
'previewHeight': 1080.toDouble()
},
},
);
plugin = CameraWindows();
cameraId = await plugin.createCamera(
const CameraDescription(
name: 'Test',
lensDirection: CameraLensDirection.back,
sensorOrientation: 0,
),
ResolutionPreset.high,
);
await plugin.initializeCamera(cameraId);
});
test('Should receive camera closing events', () async {
// Act
final Stream<CameraClosingEvent> eventStream =
plugin.onCameraClosing(cameraId);
final StreamQueue<CameraClosingEvent> streamQueue =
StreamQueue<CameraClosingEvent>(eventStream);
// Emit test events
final CameraClosingEvent event = CameraClosingEvent(cameraId);
await plugin.handleCameraMethodCall(
MethodCall('camera_closing', event.toJson()), cameraId);
await plugin.handleCameraMethodCall(
MethodCall('camera_closing', event.toJson()), cameraId);
await plugin.handleCameraMethodCall(
MethodCall('camera_closing', event.toJson()), cameraId);
// Assert
expect(await streamQueue.next, event);
expect(await streamQueue.next, event);
expect(await streamQueue.next, event);
// Clean up
await streamQueue.cancel();
});
test('Should receive camera error events', () async {
// Act
final Stream<CameraErrorEvent> errorStream =
plugin.onCameraError(cameraId);
final StreamQueue<CameraErrorEvent> streamQueue =
StreamQueue<CameraErrorEvent>(errorStream);
// Emit test events
final CameraErrorEvent event =
CameraErrorEvent(cameraId, 'Error Description');
await plugin.handleCameraMethodCall(
MethodCall('error', event.toJson()), cameraId);
await plugin.handleCameraMethodCall(
MethodCall('error', event.toJson()), cameraId);
await plugin.handleCameraMethodCall(
MethodCall('error', event.toJson()), cameraId);
// Assert
expect(await streamQueue.next, event);
expect(await streamQueue.next, event);
expect(await streamQueue.next, event);
// Clean up
await streamQueue.cancel();
});
});
group('Function Tests', () {
late CameraWindows plugin;
late int cameraId;
setUp(() async {
MethodChannelMock(
channelName: pluginChannelName,
methods: <String, dynamic>{
'create': <String, dynamic>{'cameraId': 1},
'initialize': <String, dynamic>{
'previewWidth': 1920.toDouble(),
'previewHeight': 1080.toDouble()
},
},
);
plugin = CameraWindows();
cameraId = await plugin.createCamera(
const CameraDescription(
name: 'Test',
lensDirection: CameraLensDirection.back,
sensorOrientation: 0,
),
ResolutionPreset.high,
);
await plugin.initializeCamera(cameraId);
});
test('Should fetch CameraDescription instances for available cameras',
() async {
// Arrange
final List<dynamic> returnData = <dynamic>[
<String, dynamic>{
'name': 'Test 1',
'lensFacing': 'front',
'sensorOrientation': 1
},
<String, dynamic>{
'name': 'Test 2',
'lensFacing': 'back',
'sensorOrientation': 2
}
];
final MethodChannelMock channel = MethodChannelMock(
channelName: pluginChannelName,
methods: <String, dynamic>{'availableCameras': returnData},
);
// Act
final List<CameraDescription> cameras = await plugin.availableCameras();
// Assert
expect(channel.log, <Matcher>[
isMethodCall('availableCameras', arguments: null),
]);
expect(cameras.length, returnData.length);
for (int i = 0; i < returnData.length; i++) {
final Map<String, Object?> typedData =
(returnData[i] as Map<dynamic, dynamic>).cast<String, Object?>();
final CameraDescription cameraDescription = CameraDescription(
name: typedData['name']! as String,
lensDirection: plugin
.parseCameraLensDirection(typedData['lensFacing']! as String),
sensorOrientation: typedData['sensorOrientation']! as int,
);
expect(cameras[i], cameraDescription);
}
});
test(
'Should throw CameraException when availableCameras throws a PlatformException',
() {
// Arrange
MethodChannelMock(
channelName: pluginChannelName,
methods: <String, dynamic>{
'availableCameras': PlatformException(
code: 'TESTING_ERROR_CODE',
message: 'Mock error message used during testing.',
)
});
// Act
expect(
plugin.availableCameras,
throwsA(
isA<CameraException>()
.having(
(CameraException e) => e.code, 'code', 'TESTING_ERROR_CODE')
.having((CameraException e) => e.description, 'description',
'Mock error message used during testing.'),
),
);
});
test('Should take a picture and return an XFile instance', () async {
// Arrange
final MethodChannelMock channel = MethodChannelMock(
channelName: pluginChannelName,
methods: <String, dynamic>{'takePicture': '/test/path.jpg'});
// Act
final XFile file = await plugin.takePicture(cameraId);
// Assert
expect(channel.log, <Matcher>[
isMethodCall('takePicture', arguments: <String, Object?>{
'cameraId': cameraId,
}),
]);
expect(file.path, '/test/path.jpg');
});
test('Should prepare for video recording', () async {
// Arrange
final MethodChannelMock channel = MethodChannelMock(
channelName: pluginChannelName,
methods: <String, dynamic>{'prepareForVideoRecording': null},
);
// Act
await plugin.prepareForVideoRecording();
// Assert
expect(channel.log, <Matcher>[
isMethodCall('prepareForVideoRecording', arguments: null),
]);
});
test('Should start recording a video', () async {
// Arrange
final MethodChannelMock channel = MethodChannelMock(
channelName: pluginChannelName,
methods: <String, dynamic>{'startVideoRecording': null},
);
// Act
await plugin.startVideoRecording(cameraId);
// Assert
expect(channel.log, <Matcher>[
isMethodCall('startVideoRecording', arguments: <String, Object?>{
'cameraId': cameraId,
'maxVideoDuration': null,
}),
]);
});
test('Should pass maxVideoDuration when starting recording a video',
() async {
// Arrange
final MethodChannelMock channel = MethodChannelMock(
channelName: pluginChannelName,
methods: <String, dynamic>{'startVideoRecording': null},
);
// Act
await plugin.startVideoRecording(
cameraId,
maxVideoDuration: const Duration(seconds: 10),
);
// Assert
expect(channel.log, <Matcher>[
isMethodCall('startVideoRecording', arguments: <String, Object?>{
'cameraId': cameraId,
'maxVideoDuration': 10000
}),
]);
});
test('capturing fails if trying to stream', () async {
// Act and Assert
expect(
() => plugin.startVideoCapturing(VideoCaptureOptions(cameraId,
streamCallback: (CameraImageData imageData) {})),
throwsA(isA<UnimplementedError>()),
);
});
test('Should stop a video recording and return the file', () async {
// Arrange
final MethodChannelMock channel = MethodChannelMock(
channelName: pluginChannelName,
methods: <String, dynamic>{'stopVideoRecording': '/test/path.mp4'},
);
// Act
final XFile file = await plugin.stopVideoRecording(cameraId);
// Assert
expect(channel.log, <Matcher>[
isMethodCall('stopVideoRecording', arguments: <String, Object?>{
'cameraId': cameraId,
}),
]);
expect(file.path, '/test/path.mp4');
});
test('Should throw UnsupportedError when pause video recording is called',
() async {
// Act
expect(
() => plugin.pauseVideoRecording(cameraId),
throwsA(isA<UnsupportedError>()),
);
});
test(
'Should throw UnsupportedError when resume video recording is called',
() async {
// Act
expect(
() => plugin.resumeVideoRecording(cameraId),
throwsA(isA<UnsupportedError>()),
);
});
test('Should throw UnimplementedError when flash mode is set', () async {
// Act
expect(
() => plugin.setFlashMode(cameraId, FlashMode.torch),
throwsA(isA<UnimplementedError>()),
);
});
test('Should throw UnimplementedError when exposure mode is set',
() async {
// Act
expect(
() => plugin.setExposureMode(cameraId, ExposureMode.auto),
throwsA(isA<UnimplementedError>()),
);
});
test('Should throw UnsupportedError when exposure point is set',
() async {
// Act
expect(
() => plugin.setExposurePoint(cameraId, null),
throwsA(isA<UnsupportedError>()),
);
});
test('Should get the min exposure offset', () async {
// Act
final double minExposureOffset =
await plugin.getMinExposureOffset(cameraId);
// Assert
expect(minExposureOffset, 0.0);
});
test('Should get the max exposure offset', () async {
// Act
final double maxExposureOffset =
await plugin.getMaxExposureOffset(cameraId);
// Assert
expect(maxExposureOffset, 0.0);
});
test('Should get the exposure offset step size', () async {
// Act
final double stepSize =
await plugin.getExposureOffsetStepSize(cameraId);
// Assert
expect(stepSize, 1.0);
});
test('Should throw UnimplementedError when exposure offset is set',
() async {
// Act
expect(
() => plugin.setExposureOffset(cameraId, 0.5),
throwsA(isA<UnimplementedError>()),
);
});
test('Should throw UnimplementedError when focus mode is set', () async {
// Act
expect(
() => plugin.setFocusMode(cameraId, FocusMode.auto),
throwsA(isA<UnimplementedError>()),
);
});
test('Should throw UnsupportedError when exposure point is set',
() async {
// Act
expect(
() => plugin.setFocusMode(cameraId, FocusMode.auto),
throwsA(isA<UnsupportedError>()),
);
});
test('Should build a texture widget as preview widget', () async {
// Act
final Widget widget = plugin.buildPreview(cameraId);
// Act
expect(widget is Texture, isTrue);
expect((widget as Texture).textureId, cameraId);
});
test('Should throw UnimplementedError when handling unknown method', () {
final CameraWindows plugin = CameraWindows();
expect(
() => plugin.handleCameraMethodCall(
const MethodCall('unknown_method'), 1),
throwsA(isA<UnimplementedError>()));
});
test('Should get the max zoom level', () async {
// Act
final double maxZoomLevel = await plugin.getMaxZoomLevel(cameraId);
// Assert
expect(maxZoomLevel, 1.0);
});
test('Should get the min zoom level', () async {
// Act
final double maxZoomLevel = await plugin.getMinZoomLevel(cameraId);
// Assert
expect(maxZoomLevel, 1.0);
});
test('Should throw UnimplementedError when zoom level is set', () async {
// Act
expect(
() => plugin.setZoomLevel(cameraId, 2.0),
throwsA(isA<UnimplementedError>()),
);
});
test(
'Should throw UnimplementedError when lock capture orientation is called',
() async {
// Act
expect(
() => plugin.setZoomLevel(cameraId, 2.0),
throwsA(isA<UnimplementedError>()),
);
});
test(
'Should throw UnimplementedError when unlock capture orientation is called',
() async {
// Act
expect(
() => plugin.unlockCaptureOrientation(cameraId),
throwsA(isA<UnimplementedError>()),
);
});
test('Should pause the camera preview', () async {
// Arrange
final MethodChannelMock channel = MethodChannelMock(
channelName: pluginChannelName,
methods: <String, dynamic>{'pausePreview': null},
);
// Act
await plugin.pausePreview(cameraId);
// Assert
expect(channel.log, <Matcher>[
isMethodCall('pausePreview',
arguments: <String, Object?>{'cameraId': cameraId}),
]);
});
test('Should resume the camera preview', () async {
// Arrange
final MethodChannelMock channel = MethodChannelMock(
channelName: pluginChannelName,
methods: <String, dynamic>{'resumePreview': null},
);
// Act
await plugin.resumePreview(cameraId);
// Assert
expect(channel.log, <Matcher>[
isMethodCall('resumePreview',
arguments: <String, Object?>{'cameraId': cameraId}),
]);
});
});
});
}
| plugins/packages/camera/camera_windows/test/camera_windows_test.dart/0 | {
"file_path": "plugins/packages/camera/camera_windows/test/camera_windows_test.dart",
"repo_id": "plugins",
"token_count": 9710
} | 1,201 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef PACKAGES_CAMERA_CAMERA_WINDOWS_WINDOWS_COMHEAPPTR_H_
#define PACKAGES_CAMERA_CAMERA_WINDOWS_WINDOWS_COMHEAPPTR_H_
#include <windows.h>
#include <cassert>
namespace camera_windows {
// Wrapper for COM object for automatic memory release support
// Destructor uses CoTaskMemFree to release memory allocations.
template <typename T>
class ComHeapPtr {
public:
ComHeapPtr() : p_obj_(nullptr) {}
ComHeapPtr(T* p_obj) : p_obj_(p_obj) {}
// Frees memory on destruction.
~ComHeapPtr() { Free(); }
// Prevent copying / ownership transfer as not currently needed.
ComHeapPtr(ComHeapPtr const&) = delete;
ComHeapPtr& operator=(ComHeapPtr const&) = delete;
// Returns the pointer to the memory.
operator T*() { return p_obj_; }
// Returns the pointer to the memory.
T* operator->() {
assert(p_obj_ != nullptr);
return p_obj_;
}
// Returns the pointer to the memory.
const T* operator->() const {
assert(p_obj_ != nullptr);
return p_obj_;
}
// Returns the pointer to the memory.
T** operator&() {
// Wrapped object must be nullptr to avoid memory leaks.
// Object can be released with Reset(nullptr).
assert(p_obj_ == nullptr);
return &p_obj_;
}
// Frees the memory pointed to, and sets the pointer to nullptr.
void Free() {
if (p_obj_) {
CoTaskMemFree(p_obj_);
}
p_obj_ = nullptr;
}
private:
// Pointer to memory.
T* p_obj_;
};
} // namespace camera_windows
#endif // PACKAGES_CAMERA_CAMERA_WINDOWS_WINDOWS_COMHEAPPTR_H_
| plugins/packages/camera/camera_windows/windows/com_heap_ptr.h/0 | {
"file_path": "plugins/packages/camera/camera_windows/windows/com_heap_ptr.h",
"repo_id": "plugins",
"token_count": 598
} | 1,202 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:file_selector/file_selector.dart';
import 'package:flutter/material.dart';
import 'package:path_provider/path_provider.dart';
/// Screen that shows an example of openFile
class OpenTextPage extends StatelessWidget {
/// Default Constructor
const OpenTextPage({Key? key}) : super(key: key);
Future<void> _openTextFile(BuildContext context) async {
const XTypeGroup typeGroup = XTypeGroup(
label: 'text',
extensions: <String>['txt', 'json'],
);
// This demonstrates using an initial directory for the prompt, which should
// only be done in cases where the application can likely predict where the
// file would be. In most cases, this parameter should not be provided.
final String initialDirectory =
(await getApplicationDocumentsDirectory()).path;
final XFile? file = await openFile(
acceptedTypeGroups: <XTypeGroup>[typeGroup],
initialDirectory: initialDirectory,
);
if (file == null) {
// Operation was canceled by the user.
return;
}
final String fileName = file.name;
final String fileContent = await file.readAsString();
if (context.mounted) {
await showDialog<void>(
context: context,
builder: (BuildContext context) => TextDisplay(fileName, fileContent),
);
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Open a text file'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
style: ElevatedButton.styleFrom(
// TODO(darrenaustin): Migrate to new API once it lands in stable: https://github.com/flutter/flutter/issues/105724
// ignore: deprecated_member_use
primary: Colors.blue,
// ignore: deprecated_member_use
onPrimary: Colors.white,
),
child: const Text('Press to open a text file (json, txt)'),
onPressed: () => _openTextFile(context),
),
],
),
),
);
}
}
/// Widget that displays a text file in a dialog
class TextDisplay extends StatelessWidget {
/// Default Constructor
const TextDisplay(this.fileName, this.fileContent, {Key? key})
: super(key: key);
/// File's name
final String fileName;
/// File to display
final String fileContent;
@override
Widget build(BuildContext context) {
return AlertDialog(
title: Text(fileName),
content: Scrollbar(
child: SingleChildScrollView(
child: Text(fileContent),
),
),
actions: <Widget>[
TextButton(
child: const Text('Close'),
onPressed: () => Navigator.pop(context),
),
],
);
}
}
| plugins/packages/file_selector/file_selector/example/lib/open_text_page.dart/0 | {
"file_path": "plugins/packages/file_selector/file_selector/example/lib/open_text_page.dart",
"repo_id": "plugins",
"token_count": 1182
} | 1,203 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:file_selector_platform_interface/file_selector_platform_interface.dart';
import 'src/messages.g.dart';
/// An implementation of [FileSelectorPlatform] for iOS.
class FileSelectorIOS extends FileSelectorPlatform {
final FileSelectorApi _hostApi = FileSelectorApi();
/// Registers the iOS implementation.
static void registerWith() {
FileSelectorPlatform.instance = FileSelectorIOS();
}
@override
Future<XFile?> openFile({
List<XTypeGroup>? acceptedTypeGroups,
String? initialDirectory,
String? confirmButtonText,
}) async {
final List<String> path = (await _hostApi.openFile(FileSelectorConfig(
utis: _allowedUtiListFromTypeGroups(acceptedTypeGroups),
allowMultiSelection: false)))
.cast<String>();
return path.isEmpty ? null : XFile(path.first);
}
@override
Future<List<XFile>> openFiles({
List<XTypeGroup>? acceptedTypeGroups,
String? initialDirectory,
String? confirmButtonText,
}) async {
final List<String> pathList = (await _hostApi.openFile(FileSelectorConfig(
utis: _allowedUtiListFromTypeGroups(acceptedTypeGroups),
allowMultiSelection: true)))
.cast<String>();
return pathList.map((String path) => XFile(path)).toList();
}
// Converts the type group list into a list of all allowed UTIs, since
// iOS doesn't support filter groups.
List<String> _allowedUtiListFromTypeGroups(List<XTypeGroup>? typeGroups) {
if (typeGroups == null || typeGroups.isEmpty) {
return <String>[];
}
final List<String> allowedUTIs = <String>[];
for (final XTypeGroup typeGroup in typeGroups) {
// If any group allows everything, no filtering should be done.
if (typeGroup.allowsAny) {
return <String>[];
}
if (typeGroup.macUTIs?.isEmpty ?? true) {
throw ArgumentError('The provided type group $typeGroup should either '
'allow all files, or have a non-empty "macUTIs"');
}
allowedUTIs.addAll(typeGroup.macUTIs!);
}
return allowedUTIs;
}
}
| plugins/packages/file_selector/file_selector_ios/lib/file_selector_ios.dart/0 | {
"file_path": "plugins/packages/file_selector/file_selector_ios/lib/file_selector_ios.dart",
"repo_id": "plugins",
"token_count": 811
} | 1,204 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:file_selector_platform_interface/file_selector_platform_interface.dart';
import 'package:flutter/foundation.dart' show visibleForTesting;
import 'package:flutter/services.dart';
const MethodChannel _channel =
MethodChannel('plugins.flutter.dev/file_selector_linux');
const String _typeGroupLabelKey = 'label';
const String _typeGroupExtensionsKey = 'extensions';
const String _typeGroupMimeTypesKey = 'mimeTypes';
const String _openFileMethod = 'openFile';
const String _getSavePathMethod = 'getSavePath';
const String _getDirectoryPathMethod = 'getDirectoryPath';
const String _acceptedTypeGroupsKey = 'acceptedTypeGroups';
const String _confirmButtonTextKey = 'confirmButtonText';
const String _initialDirectoryKey = 'initialDirectory';
const String _multipleKey = 'multiple';
const String _suggestedNameKey = 'suggestedName';
/// An implementation of [FileSelectorPlatform] for Linux.
class FileSelectorLinux extends FileSelectorPlatform {
/// The MethodChannel that is being used by this implementation of the plugin.
@visibleForTesting
MethodChannel get channel => _channel;
/// Registers the Linux implementation.
static void registerWith() {
FileSelectorPlatform.instance = FileSelectorLinux();
}
@override
Future<XFile?> openFile({
List<XTypeGroup>? acceptedTypeGroups,
String? initialDirectory,
String? confirmButtonText,
}) async {
final List<Map<String, Object>> serializedTypeGroups =
_serializeTypeGroups(acceptedTypeGroups);
final List<String>? path = await _channel.invokeListMethod<String>(
_openFileMethod,
<String, dynamic>{
if (serializedTypeGroups.isNotEmpty)
_acceptedTypeGroupsKey: serializedTypeGroups,
'initialDirectory': initialDirectory,
_confirmButtonTextKey: confirmButtonText,
_multipleKey: false,
},
);
return path == null ? null : XFile(path.first);
}
@override
Future<List<XFile>> openFiles({
List<XTypeGroup>? acceptedTypeGroups,
String? initialDirectory,
String? confirmButtonText,
}) async {
final List<Map<String, Object>> serializedTypeGroups =
_serializeTypeGroups(acceptedTypeGroups);
final List<String>? pathList = await _channel.invokeListMethod<String>(
_openFileMethod,
<String, dynamic>{
if (serializedTypeGroups.isNotEmpty)
_acceptedTypeGroupsKey: serializedTypeGroups,
_initialDirectoryKey: initialDirectory,
_confirmButtonTextKey: confirmButtonText,
_multipleKey: true,
},
);
return pathList?.map((String path) => XFile(path)).toList() ?? <XFile>[];
}
@override
Future<String?> getSavePath({
List<XTypeGroup>? acceptedTypeGroups,
String? initialDirectory,
String? suggestedName,
String? confirmButtonText,
}) async {
final List<Map<String, Object>> serializedTypeGroups =
_serializeTypeGroups(acceptedTypeGroups);
return _channel.invokeMethod<String>(
_getSavePathMethod,
<String, dynamic>{
if (serializedTypeGroups.isNotEmpty)
_acceptedTypeGroupsKey: serializedTypeGroups,
_initialDirectoryKey: initialDirectory,
_suggestedNameKey: suggestedName,
_confirmButtonTextKey: confirmButtonText,
},
);
}
@override
Future<String?> getDirectoryPath({
String? initialDirectory,
String? confirmButtonText,
}) async {
final List<String>? path = await _channel
.invokeListMethod<String>(_getDirectoryPathMethod, <String, dynamic>{
_initialDirectoryKey: initialDirectory,
_confirmButtonTextKey: confirmButtonText,
});
return path?.first;
}
@override
Future<List<String>> getDirectoryPaths({
String? initialDirectory,
String? confirmButtonText,
}) async {
final List<String>? pathList = await _channel
.invokeListMethod<String>(_getDirectoryPathMethod, <String, dynamic>{
_initialDirectoryKey: initialDirectory,
_confirmButtonTextKey: confirmButtonText,
_multipleKey: true,
});
return pathList ?? <String>[];
}
}
List<Map<String, Object>> _serializeTypeGroups(List<XTypeGroup>? groups) {
return (groups ?? <XTypeGroup>[]).map(_serializeTypeGroup).toList();
}
Map<String, Object> _serializeTypeGroup(XTypeGroup group) {
final Map<String, Object> serialization = <String, Object>{
_typeGroupLabelKey: group.label ?? '',
};
if (group.allowsAny) {
serialization[_typeGroupExtensionsKey] = <String>['*'];
} else {
if ((group.extensions?.isEmpty ?? true) &&
(group.mimeTypes?.isEmpty ?? true)) {
throw ArgumentError('Provided type group $group does not allow '
'all files, but does not set any of the Linux-supported filter '
'categories. "extensions" or "mimeTypes" must be non-empty for Linux '
'if anything is non-empty.');
}
if (group.extensions?.isNotEmpty ?? false) {
serialization[_typeGroupExtensionsKey] = group.extensions
?.map((String extension) => '*.$extension')
.toList() ??
<String>[];
}
if (group.mimeTypes?.isNotEmpty ?? false) {
serialization[_typeGroupMimeTypesKey] = group.mimeTypes ?? <String>[];
}
}
return serialization;
}
| plugins/packages/file_selector/file_selector_linux/lib/file_selector_linux.dart/0 | {
"file_path": "plugins/packages/file_selector/file_selector_linux/lib/file_selector_linux.dart",
"repo_id": "plugins",
"token_count": 1914
} | 1,205 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:file_selector_macos/file_selector_macos.dart';
import 'package:file_selector_macos/src/messages.g.dart';
import 'package:file_selector_platform_interface/file_selector_platform_interface.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:mockito/annotations.dart';
import 'package:mockito/mockito.dart';
import 'file_selector_macos_test.mocks.dart';
import 'messages_test.g.dart';
@GenerateMocks(<Type>[TestFileSelectorApi])
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
late FileSelectorMacOS plugin;
late MockTestFileSelectorApi mockApi;
setUp(() {
plugin = FileSelectorMacOS();
mockApi = MockTestFileSelectorApi();
TestFileSelectorApi.setup(mockApi);
// Set default stubs for tests that don't expect a specific return value,
// so calls don't throw. Tests that `expect` return values should override
// these locally.
when(mockApi.displayOpenPanel(any)).thenAnswer((_) async => <String?>[]);
when(mockApi.displaySavePanel(any)).thenAnswer((_) async => null);
});
test('registered instance', () {
FileSelectorMacOS.registerWith();
expect(FileSelectorPlatform.instance, isA<FileSelectorMacOS>());
});
group('openFile', () {
test('works as expected with no arguments', () async {
when(mockApi.displayOpenPanel(any))
.thenAnswer((_) async => <String?>['foo']);
final XFile? file = await plugin.openFile();
expect(file!.path, 'foo');
final VerificationResult result =
verify(mockApi.displayOpenPanel(captureAny));
final OpenPanelOptions options = result.captured[0] as OpenPanelOptions;
expect(options.allowsMultipleSelection, false);
expect(options.canChooseFiles, true);
expect(options.canChooseDirectories, false);
expect(options.baseOptions.allowedFileTypes, null);
expect(options.baseOptions.directoryPath, null);
expect(options.baseOptions.nameFieldStringValue, null);
expect(options.baseOptions.prompt, null);
});
test('handles cancel', () async {
when(mockApi.displayOpenPanel(any)).thenAnswer((_) async => <String?>[]);
final XFile? file = await plugin.openFile();
expect(file, null);
});
test('passes the accepted type groups correctly', () async {
const XTypeGroup group = XTypeGroup(
label: 'text',
extensions: <String>['txt'],
mimeTypes: <String>['text/plain'],
macUTIs: <String>['public.text'],
);
const XTypeGroup groupTwo = XTypeGroup(
label: 'image',
extensions: <String>['jpg'],
mimeTypes: <String>['image/jpg'],
macUTIs: <String>['public.image'],
webWildCards: <String>['image/*']);
await plugin.openFile(acceptedTypeGroups: <XTypeGroup>[group, groupTwo]);
final VerificationResult result =
verify(mockApi.displayOpenPanel(captureAny));
final OpenPanelOptions options = result.captured[0] as OpenPanelOptions;
expect(options.baseOptions.allowedFileTypes!.extensions,
<String>['txt', 'jpg']);
expect(options.baseOptions.allowedFileTypes!.mimeTypes,
<String>['text/plain', 'image/jpg']);
expect(options.baseOptions.allowedFileTypes!.utis,
<String>['public.text', 'public.image']);
});
test('passes initialDirectory correctly', () async {
await plugin.openFile(initialDirectory: '/example/directory');
final VerificationResult result =
verify(mockApi.displayOpenPanel(captureAny));
final OpenPanelOptions options = result.captured[0] as OpenPanelOptions;
expect(options.baseOptions.directoryPath, '/example/directory');
});
test('passes confirmButtonText correctly', () async {
await plugin.openFile(confirmButtonText: 'Open File');
final VerificationResult result =
verify(mockApi.displayOpenPanel(captureAny));
final OpenPanelOptions options = result.captured[0] as OpenPanelOptions;
expect(options.baseOptions.prompt, 'Open File');
});
test('throws for a type group that does not support macOS', () async {
const XTypeGroup group = XTypeGroup(
label: 'images',
webWildCards: <String>['images/*'],
);
await expectLater(
plugin.openFile(acceptedTypeGroups: <XTypeGroup>[group]),
throwsArgumentError);
});
test('allows a wildcard group', () async {
const XTypeGroup group = XTypeGroup(
label: 'text',
);
await expectLater(
plugin.openFile(acceptedTypeGroups: <XTypeGroup>[group]), completes);
});
});
group('openFiles', () {
test('works as expected with no arguments', () async {
when(mockApi.displayOpenPanel(any))
.thenAnswer((_) async => <String?>['foo', 'bar']);
final List<XFile> files = await plugin.openFiles();
expect(files[0].path, 'foo');
expect(files[1].path, 'bar');
final VerificationResult result =
verify(mockApi.displayOpenPanel(captureAny));
final OpenPanelOptions options = result.captured[0] as OpenPanelOptions;
expect(options.allowsMultipleSelection, true);
expect(options.canChooseFiles, true);
expect(options.canChooseDirectories, false);
expect(options.baseOptions.allowedFileTypes, null);
expect(options.baseOptions.directoryPath, null);
expect(options.baseOptions.nameFieldStringValue, null);
expect(options.baseOptions.prompt, null);
});
test('handles cancel', () async {
when(mockApi.displayOpenPanel(any)).thenAnswer((_) async => <String?>[]);
final List<XFile> files = await plugin.openFiles();
expect(files, isEmpty);
});
test('passes the accepted type groups correctly', () async {
const XTypeGroup group = XTypeGroup(
label: 'text',
extensions: <String>['txt'],
mimeTypes: <String>['text/plain'],
macUTIs: <String>['public.text'],
);
const XTypeGroup groupTwo = XTypeGroup(
label: 'image',
extensions: <String>['jpg'],
mimeTypes: <String>['image/jpg'],
macUTIs: <String>['public.image'],
webWildCards: <String>['image/*']);
await plugin.openFiles(acceptedTypeGroups: <XTypeGroup>[group, groupTwo]);
final VerificationResult result =
verify(mockApi.displayOpenPanel(captureAny));
final OpenPanelOptions options = result.captured[0] as OpenPanelOptions;
expect(options.baseOptions.allowedFileTypes!.extensions,
<String>['txt', 'jpg']);
expect(options.baseOptions.allowedFileTypes!.mimeTypes,
<String>['text/plain', 'image/jpg']);
expect(options.baseOptions.allowedFileTypes!.utis,
<String>['public.text', 'public.image']);
});
test('passes initialDirectory correctly', () async {
await plugin.openFiles(initialDirectory: '/example/directory');
final VerificationResult result =
verify(mockApi.displayOpenPanel(captureAny));
final OpenPanelOptions options = result.captured[0] as OpenPanelOptions;
expect(options.baseOptions.directoryPath, '/example/directory');
});
test('passes confirmButtonText correctly', () async {
await plugin.openFiles(confirmButtonText: 'Open File');
final VerificationResult result =
verify(mockApi.displayOpenPanel(captureAny));
final OpenPanelOptions options = result.captured[0] as OpenPanelOptions;
expect(options.baseOptions.prompt, 'Open File');
});
test('throws for a type group that does not support macOS', () async {
const XTypeGroup group = XTypeGroup(
label: 'images',
webWildCards: <String>['images/*'],
);
await expectLater(
plugin.openFiles(acceptedTypeGroups: <XTypeGroup>[group]),
throwsArgumentError);
});
test('allows a wildcard group', () async {
const XTypeGroup group = XTypeGroup(
label: 'text',
);
await expectLater(
plugin.openFiles(acceptedTypeGroups: <XTypeGroup>[group]), completes);
});
});
group('getSavePath', () {
test('works as expected with no arguments', () async {
when(mockApi.displaySavePanel(any)).thenAnswer((_) async => 'foo');
final String? path = await plugin.getSavePath();
expect(path, 'foo');
final VerificationResult result =
verify(mockApi.displaySavePanel(captureAny));
final SavePanelOptions options = result.captured[0] as SavePanelOptions;
expect(options.allowedFileTypes, null);
expect(options.directoryPath, null);
expect(options.nameFieldStringValue, null);
expect(options.prompt, null);
});
test('handles cancel', () async {
when(mockApi.displaySavePanel(any)).thenAnswer((_) async => null);
final String? path = await plugin.getSavePath();
expect(path, null);
});
test('passes the accepted type groups correctly', () async {
const XTypeGroup group = XTypeGroup(
label: 'text',
extensions: <String>['txt'],
mimeTypes: <String>['text/plain'],
macUTIs: <String>['public.text'],
);
const XTypeGroup groupTwo = XTypeGroup(
label: 'image',
extensions: <String>['jpg'],
mimeTypes: <String>['image/jpg'],
macUTIs: <String>['public.image'],
webWildCards: <String>['image/*']);
await plugin
.getSavePath(acceptedTypeGroups: <XTypeGroup>[group, groupTwo]);
final VerificationResult result =
verify(mockApi.displaySavePanel(captureAny));
final SavePanelOptions options = result.captured[0] as SavePanelOptions;
expect(options.allowedFileTypes!.extensions, <String>['txt', 'jpg']);
expect(options.allowedFileTypes!.mimeTypes,
<String>['text/plain', 'image/jpg']);
expect(options.allowedFileTypes!.utis,
<String>['public.text', 'public.image']);
});
test('passes initialDirectory correctly', () async {
await plugin.getSavePath(initialDirectory: '/example/directory');
final VerificationResult result =
verify(mockApi.displaySavePanel(captureAny));
final SavePanelOptions options = result.captured[0] as SavePanelOptions;
expect(options.directoryPath, '/example/directory');
});
test('passes confirmButtonText correctly', () async {
await plugin.getSavePath(confirmButtonText: 'Open File');
final VerificationResult result =
verify(mockApi.displaySavePanel(captureAny));
final SavePanelOptions options = result.captured[0] as SavePanelOptions;
expect(options.prompt, 'Open File');
});
test('throws for a type group that does not support macOS', () async {
const XTypeGroup group = XTypeGroup(
label: 'images',
webWildCards: <String>['images/*'],
);
await expectLater(
plugin.getSavePath(acceptedTypeGroups: <XTypeGroup>[group]),
throwsArgumentError);
});
test('allows a wildcard group', () async {
const XTypeGroup group = XTypeGroup(
label: 'text',
);
await expectLater(
plugin.getSavePath(acceptedTypeGroups: <XTypeGroup>[group]),
completes);
});
});
group('getDirectoryPath', () {
test('works as expected with no arguments', () async {
when(mockApi.displayOpenPanel(any))
.thenAnswer((_) async => <String?>['foo']);
final String? path = await plugin.getDirectoryPath();
expect(path, 'foo');
final VerificationResult result =
verify(mockApi.displayOpenPanel(captureAny));
final OpenPanelOptions options = result.captured[0] as OpenPanelOptions;
expect(options.allowsMultipleSelection, false);
expect(options.canChooseFiles, false);
expect(options.canChooseDirectories, true);
expect(options.baseOptions.allowedFileTypes, null);
expect(options.baseOptions.directoryPath, null);
expect(options.baseOptions.nameFieldStringValue, null);
expect(options.baseOptions.prompt, null);
});
test('handles cancel', () async {
when(mockApi.displayOpenPanel(any)).thenAnswer((_) async => <String?>[]);
final String? path = await plugin.getDirectoryPath();
expect(path, null);
});
test('passes initialDirectory correctly', () async {
await plugin.getDirectoryPath(initialDirectory: '/example/directory');
final VerificationResult result =
verify(mockApi.displayOpenPanel(captureAny));
final OpenPanelOptions options = result.captured[0] as OpenPanelOptions;
expect(options.baseOptions.directoryPath, '/example/directory');
});
test('passes confirmButtonText correctly', () async {
await plugin.getDirectoryPath(confirmButtonText: 'Open File');
final VerificationResult result =
verify(mockApi.displayOpenPanel(captureAny));
final OpenPanelOptions options = result.captured[0] as OpenPanelOptions;
expect(options.baseOptions.prompt, 'Open File');
});
});
test('ignores all type groups if any of them is a wildcard', () async {
await plugin.getSavePath(acceptedTypeGroups: <XTypeGroup>[
const XTypeGroup(
label: 'text',
extensions: <String>['txt'],
mimeTypes: <String>['text/plain'],
macUTIs: <String>['public.text'],
),
const XTypeGroup(
label: 'image',
extensions: <String>['jpg'],
mimeTypes: <String>['image/jpg'],
macUTIs: <String>['public.image'],
),
const XTypeGroup(
label: 'any',
),
]);
final VerificationResult result =
verify(mockApi.displaySavePanel(captureAny));
final SavePanelOptions options = result.captured[0] as SavePanelOptions;
expect(options.allowedFileTypes, null);
});
}
| plugins/packages/file_selector/file_selector_macos/test/file_selector_macos_test.dart/0 | {
"file_path": "plugins/packages/file_selector/file_selector_macos/test/file_selector_macos_test.dart",
"repo_id": "plugins",
"token_count": 5296
} | 1,206 |
name: file_selector_web
description: Web platform implementation of file_selector
repository: https://github.com/flutter/plugins/tree/main/packages/file_selector/file_selector_web
issue_tracker: https://github.com/flutter/flutter/issues?q=is%3Aissue+is%3Aopen+label%3A%22p%3A+file_selector%22
version: 0.9.0+2
environment:
sdk: ">=2.12.0 <3.0.0"
flutter: ">=3.0.0"
flutter:
plugin:
implements: file_selector
platforms:
web:
pluginClass: FileSelectorWeb
fileName: file_selector_web.dart
dependencies:
file_selector_platform_interface: ^2.2.0
flutter:
sdk: flutter
flutter_web_plugins:
sdk: flutter
dev_dependencies:
flutter_test:
sdk: flutter
| plugins/packages/file_selector/file_selector_web/pubspec.yaml/0 | {
"file_path": "plugins/packages/file_selector/file_selector_web/pubspec.yaml",
"repo_id": "plugins",
"token_count": 297
} | 1,207 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:typed_data';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:google_maps_flutter/google_maps_flutter.dart';
class FakePlatformGoogleMap {
FakePlatformGoogleMap(int id, Map<dynamic, dynamic> params)
: cameraPosition =
CameraPosition.fromMap(params['initialCameraPosition']),
channel = MethodChannel('plugins.flutter.io/google_maps_$id') {
_ambiguate(TestDefaultBinaryMessengerBinding.instance)!
.defaultBinaryMessenger
.setMockMethodCallHandler(channel, onMethodCall);
updateOptions(params['options'] as Map<dynamic, dynamic>);
updateMarkers(params);
updatePolygons(params);
updatePolylines(params);
updateCircles(params);
updateTileOverlays(Map.castFrom<dynamic, dynamic, String, dynamic>(params));
}
MethodChannel channel;
CameraPosition? cameraPosition;
bool? compassEnabled;
bool? mapToolbarEnabled;
CameraTargetBounds? cameraTargetBounds;
MapType? mapType;
MinMaxZoomPreference? minMaxZoomPreference;
bool? rotateGesturesEnabled;
bool? scrollGesturesEnabled;
bool? tiltGesturesEnabled;
bool? zoomGesturesEnabled;
bool? zoomControlsEnabled;
bool? liteModeEnabled;
bool? trackCameraPosition;
bool? myLocationEnabled;
bool? trafficEnabled;
bool? buildingsEnabled;
bool? myLocationButtonEnabled;
List<dynamic>? padding;
Set<MarkerId> markerIdsToRemove = <MarkerId>{};
Set<Marker> markersToAdd = <Marker>{};
Set<Marker> markersToChange = <Marker>{};
Set<PolygonId> polygonIdsToRemove = <PolygonId>{};
Set<Polygon> polygonsToAdd = <Polygon>{};
Set<Polygon> polygonsToChange = <Polygon>{};
Set<PolylineId> polylineIdsToRemove = <PolylineId>{};
Set<Polyline> polylinesToAdd = <Polyline>{};
Set<Polyline> polylinesToChange = <Polyline>{};
Set<CircleId> circleIdsToRemove = <CircleId>{};
Set<Circle> circlesToAdd = <Circle>{};
Set<Circle> circlesToChange = <Circle>{};
Set<TileOverlayId> tileOverlayIdsToRemove = <TileOverlayId>{};
Set<TileOverlay> tileOverlaysToAdd = <TileOverlay>{};
Set<TileOverlay> tileOverlaysToChange = <TileOverlay>{};
Future<dynamic> onMethodCall(MethodCall call) {
switch (call.method) {
case 'map#update':
final Map<String, Object?> arguments =
(call.arguments as Map<Object?, Object?>).cast<String, Object?>();
updateOptions(arguments['options']! as Map<dynamic, dynamic>);
return Future<void>.sync(() {});
case 'markers#update':
updateMarkers(call.arguments as Map<dynamic, dynamic>?);
return Future<void>.sync(() {});
case 'polygons#update':
updatePolygons(call.arguments as Map<dynamic, dynamic>?);
return Future<void>.sync(() {});
case 'polylines#update':
updatePolylines(call.arguments as Map<dynamic, dynamic>?);
return Future<void>.sync(() {});
case 'tileOverlays#update':
updateTileOverlays(Map.castFrom<dynamic, dynamic, String, dynamic>(
call.arguments as Map<dynamic, dynamic>));
return Future<void>.sync(() {});
case 'circles#update':
updateCircles(call.arguments as Map<dynamic, dynamic>?);
return Future<void>.sync(() {});
default:
return Future<void>.sync(() {});
}
}
void updateMarkers(Map<dynamic, dynamic>? markerUpdates) {
if (markerUpdates == null) {
return;
}
markersToAdd = _deserializeMarkers(markerUpdates['markersToAdd']);
markerIdsToRemove = _deserializeMarkerIds(
markerUpdates['markerIdsToRemove'] as List<dynamic>?);
markersToChange = _deserializeMarkers(markerUpdates['markersToChange']);
}
Set<MarkerId> _deserializeMarkerIds(List<dynamic>? markerIds) {
if (markerIds == null) {
return <MarkerId>{};
}
return markerIds
.map((dynamic markerId) => MarkerId(markerId as String))
.toSet();
}
Set<Marker> _deserializeMarkers(dynamic markers) {
if (markers == null) {
return <Marker>{};
}
final List<dynamic> markersData = markers as List<dynamic>;
final Set<Marker> result = <Marker>{};
for (final Map<dynamic, dynamic> markerData
in markersData.cast<Map<dynamic, dynamic>>()) {
final String markerId = markerData['markerId'] as String;
final double alpha = markerData['alpha'] as double;
final bool draggable = markerData['draggable'] as bool;
final bool visible = markerData['visible'] as bool;
final dynamic infoWindowData = markerData['infoWindow'];
InfoWindow infoWindow = InfoWindow.noText;
if (infoWindowData != null) {
final Map<dynamic, dynamic> infoWindowMap =
infoWindowData as Map<dynamic, dynamic>;
infoWindow = InfoWindow(
title: infoWindowMap['title'] as String?,
snippet: infoWindowMap['snippet'] as String?,
);
}
result.add(Marker(
markerId: MarkerId(markerId),
draggable: draggable,
visible: visible,
infoWindow: infoWindow,
alpha: alpha,
));
}
return result;
}
void updatePolygons(Map<dynamic, dynamic>? polygonUpdates) {
if (polygonUpdates == null) {
return;
}
polygonsToAdd = _deserializePolygons(polygonUpdates['polygonsToAdd']);
polygonIdsToRemove = _deserializePolygonIds(
polygonUpdates['polygonIdsToRemove'] as List<dynamic>?);
polygonsToChange = _deserializePolygons(polygonUpdates['polygonsToChange']);
}
Set<PolygonId> _deserializePolygonIds(List<dynamic>? polygonIds) {
if (polygonIds == null) {
return <PolygonId>{};
}
return polygonIds
.map((dynamic polygonId) => PolygonId(polygonId as String))
.toSet();
}
Set<Polygon> _deserializePolygons(dynamic polygons) {
if (polygons == null) {
return <Polygon>{};
}
final List<dynamic> polygonsData = polygons as List<dynamic>;
final Set<Polygon> result = <Polygon>{};
for (final Map<dynamic, dynamic> polygonData
in polygonsData.cast<Map<dynamic, dynamic>>()) {
final String polygonId = polygonData['polygonId'] as String;
final bool visible = polygonData['visible'] as bool;
final bool geodesic = polygonData['geodesic'] as bool;
final List<LatLng> points =
_deserializePoints(polygonData['points'] as List<dynamic>);
final List<List<LatLng>> holes =
_deserializeHoles(polygonData['holes'] as List<dynamic>);
result.add(Polygon(
polygonId: PolygonId(polygonId),
visible: visible,
geodesic: geodesic,
points: points,
holes: holes,
));
}
return result;
}
// Converts a list of points expressed as two-element lists of doubles into
// a list of `LatLng`s. All list items are assumed to be non-null.
List<LatLng> _deserializePoints(List<dynamic> points) {
return points.map<LatLng>((dynamic item) {
final List<Object?> list = item as List<Object?>;
return LatLng(list[0]! as double, list[1]! as double);
}).toList();
}
List<List<LatLng>> _deserializeHoles(List<dynamic> holes) {
return holes.map<List<LatLng>>((dynamic hole) {
return _deserializePoints(hole as List<dynamic>);
}).toList();
}
void updatePolylines(Map<dynamic, dynamic>? polylineUpdates) {
if (polylineUpdates == null) {
return;
}
polylinesToAdd = _deserializePolylines(polylineUpdates['polylinesToAdd']);
polylineIdsToRemove = _deserializePolylineIds(
polylineUpdates['polylineIdsToRemove'] as List<dynamic>?);
polylinesToChange =
_deserializePolylines(polylineUpdates['polylinesToChange']);
}
Set<PolylineId> _deserializePolylineIds(List<dynamic>? polylineIds) {
if (polylineIds == null) {
return <PolylineId>{};
}
return polylineIds
.map((dynamic polylineId) => PolylineId(polylineId as String))
.toSet();
}
Set<Polyline> _deserializePolylines(dynamic polylines) {
if (polylines == null) {
return <Polyline>{};
}
final List<dynamic> polylinesData = polylines as List<dynamic>;
final Set<Polyline> result = <Polyline>{};
for (final Map<dynamic, dynamic> polylineData
in polylinesData.cast<Map<dynamic, dynamic>>()) {
final String polylineId = polylineData['polylineId'] as String;
final bool visible = polylineData['visible'] as bool;
final bool geodesic = polylineData['geodesic'] as bool;
final List<LatLng> points =
_deserializePoints(polylineData['points'] as List<dynamic>);
result.add(Polyline(
polylineId: PolylineId(polylineId),
visible: visible,
geodesic: geodesic,
points: points,
));
}
return result;
}
void updateCircles(Map<dynamic, dynamic>? circleUpdates) {
if (circleUpdates == null) {
return;
}
circlesToAdd = _deserializeCircles(circleUpdates['circlesToAdd']);
circleIdsToRemove = _deserializeCircleIds(
circleUpdates['circleIdsToRemove'] as List<dynamic>?);
circlesToChange = _deserializeCircles(circleUpdates['circlesToChange']);
}
void updateTileOverlays(Map<String, dynamic> updateTileOverlayUpdates) {
if (updateTileOverlayUpdates == null) {
return;
}
final List<Map<dynamic, dynamic>>? tileOverlaysToAddList =
updateTileOverlayUpdates['tileOverlaysToAdd'] != null
? List.castFrom<dynamic, Map<dynamic, dynamic>>(
updateTileOverlayUpdates['tileOverlaysToAdd'] as List<dynamic>)
: null;
final List<String>? tileOverlayIdsToRemoveList =
updateTileOverlayUpdates['tileOverlayIdsToRemove'] != null
? List.castFrom<dynamic, String>(
updateTileOverlayUpdates['tileOverlayIdsToRemove']
as List<dynamic>)
: null;
final List<Map<dynamic, dynamic>>? tileOverlaysToChangeList =
updateTileOverlayUpdates['tileOverlaysToChange'] != null
? List.castFrom<dynamic, Map<dynamic, dynamic>>(
updateTileOverlayUpdates['tileOverlaysToChange']
as List<dynamic>)
: null;
tileOverlaysToAdd = _deserializeTileOverlays(tileOverlaysToAddList);
tileOverlayIdsToRemove =
_deserializeTileOverlayIds(tileOverlayIdsToRemoveList);
tileOverlaysToChange = _deserializeTileOverlays(tileOverlaysToChangeList);
}
Set<CircleId> _deserializeCircleIds(List<dynamic>? circleIds) {
if (circleIds == null) {
return <CircleId>{};
}
return circleIds
.map((dynamic circleId) => CircleId(circleId as String))
.toSet();
}
Set<Circle> _deserializeCircles(dynamic circles) {
if (circles == null) {
return <Circle>{};
}
final List<dynamic> circlesData = circles as List<dynamic>;
final Set<Circle> result = <Circle>{};
for (final Map<dynamic, dynamic> circleData
in circlesData.cast<Map<dynamic, dynamic>>()) {
final String circleId = circleData['circleId'] as String;
final bool visible = circleData['visible'] as bool;
final double radius = circleData['radius'] as double;
result.add(Circle(
circleId: CircleId(circleId),
visible: visible,
radius: radius,
));
}
return result;
}
Set<TileOverlayId> _deserializeTileOverlayIds(List<String>? tileOverlayIds) {
if (tileOverlayIds == null || tileOverlayIds.isEmpty) {
return <TileOverlayId>{};
}
return tileOverlayIds
.map((String tileOverlayId) => TileOverlayId(tileOverlayId))
.toSet();
}
Set<TileOverlay> _deserializeTileOverlays(
List<Map<dynamic, dynamic>>? tileOverlays) {
if (tileOverlays == null || tileOverlays.isEmpty) {
return <TileOverlay>{};
}
final Set<TileOverlay> result = <TileOverlay>{};
for (final Map<dynamic, dynamic> tileOverlayData in tileOverlays) {
final String tileOverlayId = tileOverlayData['tileOverlayId'] as String;
final bool fadeIn = tileOverlayData['fadeIn'] as bool;
final double transparency = tileOverlayData['transparency'] as double;
final int zIndex = tileOverlayData['zIndex'] as int;
final bool visible = tileOverlayData['visible'] as bool;
result.add(TileOverlay(
tileOverlayId: TileOverlayId(tileOverlayId),
fadeIn: fadeIn,
transparency: transparency,
zIndex: zIndex,
visible: visible,
));
}
return result;
}
void updateOptions(Map<dynamic, dynamic> options) {
if (options.containsKey('compassEnabled')) {
compassEnabled = options['compassEnabled'] as bool?;
}
if (options.containsKey('mapToolbarEnabled')) {
mapToolbarEnabled = options['mapToolbarEnabled'] as bool?;
}
if (options.containsKey('cameraTargetBounds')) {
final List<dynamic> boundsList =
options['cameraTargetBounds'] as List<dynamic>;
cameraTargetBounds = boundsList[0] == null
? CameraTargetBounds.unbounded
: CameraTargetBounds(LatLngBounds.fromList(boundsList[0]));
}
if (options.containsKey('mapType')) {
mapType = MapType.values[options['mapType'] as int];
}
if (options.containsKey('minMaxZoomPreference')) {
final List<dynamic> minMaxZoomList =
options['minMaxZoomPreference'] as List<dynamic>;
minMaxZoomPreference = MinMaxZoomPreference(
minMaxZoomList[0] as double?, minMaxZoomList[1] as double?);
}
if (options.containsKey('rotateGesturesEnabled')) {
rotateGesturesEnabled = options['rotateGesturesEnabled'] as bool?;
}
if (options.containsKey('scrollGesturesEnabled')) {
scrollGesturesEnabled = options['scrollGesturesEnabled'] as bool?;
}
if (options.containsKey('tiltGesturesEnabled')) {
tiltGesturesEnabled = options['tiltGesturesEnabled'] as bool?;
}
if (options.containsKey('trackCameraPosition')) {
trackCameraPosition = options['trackCameraPosition'] as bool?;
}
if (options.containsKey('zoomGesturesEnabled')) {
zoomGesturesEnabled = options['zoomGesturesEnabled'] as bool?;
}
if (options.containsKey('zoomControlsEnabled')) {
zoomControlsEnabled = options['zoomControlsEnabled'] as bool?;
}
if (options.containsKey('liteModeEnabled')) {
liteModeEnabled = options['liteModeEnabled'] as bool?;
}
if (options.containsKey('myLocationEnabled')) {
myLocationEnabled = options['myLocationEnabled'] as bool?;
}
if (options.containsKey('myLocationButtonEnabled')) {
myLocationButtonEnabled = options['myLocationButtonEnabled'] as bool?;
}
if (options.containsKey('trafficEnabled')) {
trafficEnabled = options['trafficEnabled'] as bool?;
}
if (options.containsKey('buildingsEnabled')) {
buildingsEnabled = options['buildingsEnabled'] as bool?;
}
if (options.containsKey('padding')) {
padding = options['padding'] as List<dynamic>?;
}
}
}
class FakePlatformViewsController {
FakePlatformGoogleMap? lastCreatedView;
Future<dynamic> fakePlatformViewsMethodHandler(MethodCall call) {
switch (call.method) {
case 'create':
final Map<dynamic, dynamic> args =
call.arguments as Map<dynamic, dynamic>;
final Map<dynamic, dynamic> params =
_decodeParams(args['params'] as Uint8List)!;
lastCreatedView = FakePlatformGoogleMap(
args['id'] as int,
params,
);
return Future<int>.sync(() => 1);
default:
return Future<void>.sync(() {});
}
}
void reset() {
lastCreatedView = null;
}
}
Map<dynamic, dynamic>? _decodeParams(Uint8List paramsMessage) {
final ByteBuffer buffer = paramsMessage.buffer;
final ByteData messageBytes = buffer.asByteData(
paramsMessage.offsetInBytes,
paramsMessage.lengthInBytes,
);
return const StandardMessageCodec().decodeMessage(messageBytes)
as Map<dynamic, dynamic>?;
}
/// This allows a value of type T or T? to be treated as a value of type T?.
///
/// We use this so that APIs that have become non-nullable can still be used
/// with `!` and `?` on the stable branch.
T? _ambiguate<T>(T? value) => value;
| plugins/packages/google_maps_flutter/google_maps_flutter/test/fake_maps_controllers.dart/0 | {
"file_path": "plugins/packages/google_maps_flutter/google_maps_flutter/test/fake_maps_controllers.dart",
"repo_id": "plugins",
"token_count": 6525
} | 1,208 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
@import GoogleMaps;
/**
* Defines a map view used for testing key-value observing.
*/
@interface PartiallyMockedMapView : GMSMapView
/**
* The number of times that the `frame` KVO has been added.
*/
@property(nonatomic, assign, readonly) NSInteger frameObserverCount;
@end
| plugins/packages/google_maps_flutter/google_maps_flutter_ios/example/ios/RunnerTests/PartiallyMockedMapView.h/0 | {
"file_path": "plugins/packages/google_maps_flutter/google_maps_flutter_ios/example/ios/RunnerTests/PartiallyMockedMapView.h",
"repo_id": "plugins",
"token_count": 127
} | 1,209 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#import "GoogleMapCircleController.h"
#import "FLTGoogleMapJSONConversions.h"
@interface FLTGoogleMapCircleController ()
@property(nonatomic, strong) GMSCircle *circle;
@property(nonatomic, weak) GMSMapView *mapView;
@end
@implementation FLTGoogleMapCircleController
- (instancetype)initCircleWithPosition:(CLLocationCoordinate2D)position
radius:(CLLocationDistance)radius
circleId:(NSString *)circleIdentifier
mapView:(GMSMapView *)mapView
options:(NSDictionary *)options {
self = [super init];
if (self) {
_circle = [GMSCircle circleWithPosition:position radius:radius];
_mapView = mapView;
_circle.userData = @[ circleIdentifier ];
[self interpretCircleOptions:options];
}
return self;
}
- (void)removeCircle {
self.circle.map = nil;
}
- (void)setConsumeTapEvents:(BOOL)consumes {
self.circle.tappable = consumes;
}
- (void)setVisible:(BOOL)visible {
self.circle.map = visible ? self.mapView : nil;
}
- (void)setZIndex:(int)zIndex {
self.circle.zIndex = zIndex;
}
- (void)setCenter:(CLLocationCoordinate2D)center {
self.circle.position = center;
}
- (void)setRadius:(CLLocationDistance)radius {
self.circle.radius = radius;
}
- (void)setStrokeColor:(UIColor *)color {
self.circle.strokeColor = color;
}
- (void)setStrokeWidth:(CGFloat)width {
self.circle.strokeWidth = width;
}
- (void)setFillColor:(UIColor *)color {
self.circle.fillColor = color;
}
- (void)interpretCircleOptions:(NSDictionary *)data {
NSNumber *consumeTapEvents = data[@"consumeTapEvents"];
if (consumeTapEvents && consumeTapEvents != (id)[NSNull null]) {
[self setConsumeTapEvents:consumeTapEvents.boolValue];
}
NSNumber *visible = data[@"visible"];
if (visible && visible != (id)[NSNull null]) {
[self setVisible:[visible boolValue]];
}
NSNumber *zIndex = data[@"zIndex"];
if (zIndex && zIndex != (id)[NSNull null]) {
[self setZIndex:[zIndex intValue]];
}
NSArray *center = data[@"center"];
if (center && center != (id)[NSNull null]) {
[self setCenter:[FLTGoogleMapJSONConversions locationFromLatLong:center]];
}
NSNumber *radius = data[@"radius"];
if (radius && radius != (id)[NSNull null]) {
[self setRadius:[radius floatValue]];
}
NSNumber *strokeColor = data[@"strokeColor"];
if (strokeColor && strokeColor != (id)[NSNull null]) {
[self setStrokeColor:[FLTGoogleMapJSONConversions colorFromRGBA:strokeColor]];
}
NSNumber *strokeWidth = data[@"strokeWidth"];
if (strokeWidth && strokeWidth != (id)[NSNull null]) {
[self setStrokeWidth:[strokeWidth intValue]];
}
NSNumber *fillColor = data[@"fillColor"];
if (fillColor && fillColor != (id)[NSNull null]) {
[self setFillColor:[FLTGoogleMapJSONConversions colorFromRGBA:fillColor]];
}
}
@end
@interface FLTCirclesController ()
@property(strong, nonatomic) FlutterMethodChannel *methodChannel;
@property(weak, nonatomic) GMSMapView *mapView;
@property(strong, nonatomic) NSMutableDictionary *circleIdToController;
@end
@implementation FLTCirclesController
- (instancetype)init:(FlutterMethodChannel *)methodChannel
mapView:(GMSMapView *)mapView
registrar:(NSObject<FlutterPluginRegistrar> *)registrar {
self = [super init];
if (self) {
_methodChannel = methodChannel;
_mapView = mapView;
_circleIdToController = [NSMutableDictionary dictionaryWithCapacity:1];
}
return self;
}
- (void)addCircles:(NSArray *)circlesToAdd {
for (NSDictionary *circle in circlesToAdd) {
CLLocationCoordinate2D position = [FLTCirclesController getPosition:circle];
CLLocationDistance radius = [FLTCirclesController getRadius:circle];
NSString *circleId = [FLTCirclesController getCircleId:circle];
FLTGoogleMapCircleController *controller =
[[FLTGoogleMapCircleController alloc] initCircleWithPosition:position
radius:radius
circleId:circleId
mapView:self.mapView
options:circle];
self.circleIdToController[circleId] = controller;
}
}
- (void)changeCircles:(NSArray *)circlesToChange {
for (NSDictionary *circle in circlesToChange) {
NSString *circleId = [FLTCirclesController getCircleId:circle];
FLTGoogleMapCircleController *controller = self.circleIdToController[circleId];
if (!controller) {
continue;
}
[controller interpretCircleOptions:circle];
}
}
- (void)removeCircleWithIdentifiers:(NSArray *)identifiers {
for (NSString *identifier in identifiers) {
FLTGoogleMapCircleController *controller = self.circleIdToController[identifier];
if (!controller) {
continue;
}
[controller removeCircle];
[self.circleIdToController removeObjectForKey:identifier];
}
}
- (bool)hasCircleWithIdentifier:(NSString *)identifier {
if (!identifier) {
return false;
}
return self.circleIdToController[identifier] != nil;
}
- (void)didTapCircleWithIdentifier:(NSString *)identifier {
if (!identifier) {
return;
}
FLTGoogleMapCircleController *controller = self.circleIdToController[identifier];
if (!controller) {
return;
}
[self.methodChannel invokeMethod:@"circle#onTap" arguments:@{@"circleId" : identifier}];
}
+ (CLLocationCoordinate2D)getPosition:(NSDictionary *)circle {
NSArray *center = circle[@"center"];
return [FLTGoogleMapJSONConversions locationFromLatLong:center];
}
+ (CLLocationDistance)getRadius:(NSDictionary *)circle {
NSNumber *radius = circle[@"radius"];
return [radius floatValue];
}
+ (NSString *)getCircleId:(NSDictionary *)circle {
return circle[@"circleId"];
}
@end
| plugins/packages/google_maps_flutter/google_maps_flutter_ios/ios/Classes/GoogleMapCircleController.m/0 | {
"file_path": "plugins/packages/google_maps_flutter/google_maps_flutter_ios/ios/Classes/GoogleMapCircleController.m",
"repo_id": "plugins",
"token_count": 2314
} | 1,210 |
name: google_maps_flutter_ios
description: iOS implementation of the google_maps_flutter plugin.
repository: https://github.com/flutter/plugins/tree/main/packages/google_maps_flutter/google_maps_flutter_ios
issue_tracker: https://github.com/flutter/flutter/issues?q=is%3Aissue+is%3Aopen+label%3A%22p%3A+maps%22
version: 2.1.13
environment:
sdk: ">=2.14.0 <3.0.0"
flutter: ">=3.0.0"
flutter:
plugin:
implements: google_maps_flutter
platforms:
ios:
pluginClass: FLTGoogleMapsPlugin
dartPluginClass: GoogleMapsFlutterIOS
dependencies:
flutter:
sdk: flutter
google_maps_flutter_platform_interface: ^2.2.1
stream_transform: ^2.0.0
dev_dependencies:
async: ^2.5.0
flutter_test:
sdk: flutter
plugin_platform_interface: ^2.0.0
| plugins/packages/google_maps_flutter/google_maps_flutter_ios/pubspec.yaml/0 | {
"file_path": "plugins/packages/google_maps_flutter/google_maps_flutter_ios/pubspec.yaml",
"repo_id": "plugins",
"token_count": 324
} | 1,211 |
# google_maps_flutter_web
This is an implementation of the [google_maps_flutter](https://pub.dev/packages/google_maps_flutter) plugin for web. Behind the scenes, it uses a14n's [google_maps](https://pub.dev/packages/google_maps) dart JS interop layer.
## Usage
### Depend on the package
This package is not an endorsed implementation of the google_maps_flutter plugin yet, so you'll need to
[add it explicitly](https://pub.dev/packages/google_maps_flutter_web/install).
### Modify web/index.html
Get an API Key for Google Maps JavaScript API. Get started [here](https://developers.google.com/maps/documentation/javascript/get-api-key).
Modify the `<head>` tag of your `web/index.html` to load the Google Maps JavaScript API, like so:
```html
<head>
<!-- // Other stuff -->
<script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY"></script>
</head>
```
Now you should be able to use the Google Maps plugin normally.
## Limitations of the web version
The following map options are not available in web, because the map doesn't rotate there:
* `compassEnabled`
* `rotateGesturesEnabled`
* `tiltGesturesEnabled`
There's no "Map Toolbar" in web, so the `mapToolbarEnabled` option is unused.
There's no "My Location" widget in web ([tracking issue](https://github.com/flutter/flutter/issues/64073)), so the following options are ignored, for now:
* `myLocationButtonEnabled`
* `myLocationEnabled`
There's no `defaultMarkerWithHue` in web. If you need colored pins/markers, you may need to use your own asset images.
Indoor and building layers are still not available on the web. Traffic is.
Only Android supports "[Lite Mode](https://developers.google.com/maps/documentation/android-sdk/lite)", so the `liteModeEnabled` constructor argument can't be set to `true` on web apps.
Google Maps for web uses `HtmlElementView` to render maps. When a `GoogleMap` is stacked below other widgets, [`package:pointer_interceptor`](https://www.pub.dev/packages/pointer_interceptor) must be used to capture mouse events on the Flutter overlays. See issue [#73830](https://github.com/flutter/flutter/issues/73830).
| plugins/packages/google_maps_flutter/google_maps_flutter_web/README.md/0 | {
"file_path": "plugins/packages/google_maps_flutter/google_maps_flutter_web/README.md",
"repo_id": "plugins",
"token_count": 631
} | 1,212 |
// Mocks generated by Mockito 5.1.0 from annotations
// in google_sign_in/test/google_sign_in_test.dart.
// Do not manually edit this file.
import 'dart:async' as _i4;
import 'package:google_sign_in_platform_interface/google_sign_in_platform_interface.dart'
as _i3;
import 'package:google_sign_in_platform_interface/src/types.dart' as _i2;
import 'package:mockito/mockito.dart' as _i1;
// ignore_for_file: type=lint
// ignore_for_file: avoid_redundant_argument_values
// ignore_for_file: avoid_setters_without_getters
// ignore_for_file: comment_references
// ignore_for_file: implementation_imports
// ignore_for_file: invalid_use_of_visible_for_testing_member
// ignore_for_file: prefer_const_constructors
// ignore_for_file: unnecessary_parenthesis
// ignore_for_file: camel_case_types
class _FakeGoogleSignInTokenData_0 extends _i1.Fake
implements _i2.GoogleSignInTokenData {}
/// A class which mocks [GoogleSignInPlatform].
///
/// See the documentation for Mockito's code generation for more information.
class MockGoogleSignInPlatform extends _i1.Mock
implements _i3.GoogleSignInPlatform {
MockGoogleSignInPlatform() {
_i1.throwOnMissingStub(this);
}
@override
bool get isMock =>
(super.noSuchMethod(Invocation.getter(#isMock), returnValue: false)
as bool);
@override
_i4.Future<void> init(
{List<String>? scopes = const [],
_i2.SignInOption? signInOption = _i2.SignInOption.standard,
String? hostedDomain,
String? clientId}) =>
(super.noSuchMethod(
Invocation.method(#init, [], {
#scopes: scopes,
#signInOption: signInOption,
#hostedDomain: hostedDomain,
#clientId: clientId
}),
returnValue: Future<void>.value(),
returnValueForMissingStub: Future<void>.value()) as _i4.Future<void>);
@override
_i4.Future<void> initWithParams(_i2.SignInInitParameters? params) =>
(super.noSuchMethod(Invocation.method(#initWithParams, [params]),
returnValue: Future<void>.value(),
returnValueForMissingStub: Future<void>.value()) as _i4.Future<void>);
@override
_i4.Future<_i2.GoogleSignInUserData?> signInSilently() =>
(super.noSuchMethod(Invocation.method(#signInSilently, []),
returnValue: Future<_i2.GoogleSignInUserData?>.value())
as _i4.Future<_i2.GoogleSignInUserData?>);
@override
_i4.Future<_i2.GoogleSignInUserData?> signIn() =>
(super.noSuchMethod(Invocation.method(#signIn, []),
returnValue: Future<_i2.GoogleSignInUserData?>.value())
as _i4.Future<_i2.GoogleSignInUserData?>);
@override
_i4.Future<_i2.GoogleSignInTokenData> getTokens(
{String? email, bool? shouldRecoverAuth}) =>
(super.noSuchMethod(
Invocation.method(#getTokens, [],
{#email: email, #shouldRecoverAuth: shouldRecoverAuth}),
returnValue: Future<_i2.GoogleSignInTokenData>.value(
_FakeGoogleSignInTokenData_0()))
as _i4.Future<_i2.GoogleSignInTokenData>);
@override
_i4.Future<void> signOut() =>
(super.noSuchMethod(Invocation.method(#signOut, []),
returnValue: Future<void>.value(),
returnValueForMissingStub: Future<void>.value()) as _i4.Future<void>);
@override
_i4.Future<void> disconnect() =>
(super.noSuchMethod(Invocation.method(#disconnect, []),
returnValue: Future<void>.value(),
returnValueForMissingStub: Future<void>.value()) as _i4.Future<void>);
@override
_i4.Future<bool> isSignedIn() =>
(super.noSuchMethod(Invocation.method(#isSignedIn, []),
returnValue: Future<bool>.value(false)) as _i4.Future<bool>);
@override
_i4.Future<void> clearAuthCache({String? token}) => (super.noSuchMethod(
Invocation.method(#clearAuthCache, [], {#token: token}),
returnValue: Future<void>.value(),
returnValueForMissingStub: Future<void>.value()) as _i4.Future<void>);
@override
_i4.Future<bool> requestScopes(List<String>? scopes) =>
(super.noSuchMethod(Invocation.method(#requestScopes, [scopes]),
returnValue: Future<bool>.value(false)) as _i4.Future<bool>);
}
| plugins/packages/google_sign_in/google_sign_in/test/google_sign_in_test.mocks.dart/0 | {
"file_path": "plugins/packages/google_sign_in/google_sign_in/test/google_sign_in_test.mocks.dart",
"repo_id": "plugins",
"token_count": 1737
} | 1,213 |
org.gradle.jvmargs=-Xmx4G
android.enableR8=true
android.useAndroidX=true
| plugins/packages/google_sign_in/google_sign_in_android/example/android/gradle.properties/0 | {
"file_path": "plugins/packages/google_sign_in/google_sign_in_android/example/android/gradle.properties",
"repo_id": "plugins",
"token_count": 30
} | 1,214 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// ignore_for_file: deprecated_member_use_from_same_package
// This file preserves the tests for the deprecated methods as they were before
// the migration. See image_picker_test.dart for the current tests.
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:image_picker/image_picker.dart';
import 'package:image_picker_platform_interface/image_picker_platform_interface.dart';
import 'package:mockito/mockito.dart';
import 'package:plugin_platform_interface/plugin_platform_interface.dart';
import 'image_picker_test.mocks.dart' as base_mock;
// Add the mixin to make the platform interface accept the mock.
class MockImagePickerPlatform extends base_mock.MockImagePickerPlatform
with MockPlatformInterfaceMixin {}
void main() {
group('ImagePicker', () {
late MockImagePickerPlatform mockPlatform;
setUp(() {
mockPlatform = MockImagePickerPlatform();
ImagePickerPlatform.instance = mockPlatform;
});
group('#Single image/video', () {
setUp(() {
when(mockPlatform.pickImage(
source: anyNamed('source'),
maxWidth: anyNamed('maxWidth'),
maxHeight: anyNamed('maxHeight'),
imageQuality: anyNamed('imageQuality'),
preferredCameraDevice: anyNamed('preferredCameraDevice')))
.thenAnswer((Invocation _) async => null);
});
group('#pickImage', () {
test('passes the image source argument correctly', () async {
final ImagePicker picker = ImagePicker();
await picker.getImage(source: ImageSource.camera);
await picker.getImage(source: ImageSource.gallery);
verifyInOrder(<Object>[
mockPlatform.pickImage(source: ImageSource.camera),
mockPlatform.pickImage(source: ImageSource.gallery),
]);
});
test('passes the width and height arguments correctly', () async {
final ImagePicker picker = ImagePicker();
await picker.getImage(source: ImageSource.camera);
await picker.getImage(
source: ImageSource.camera,
maxWidth: 10.0,
);
await picker.getImage(
source: ImageSource.camera,
maxHeight: 10.0,
);
await picker.getImage(
source: ImageSource.camera,
maxWidth: 10.0,
maxHeight: 20.0,
);
await picker.getImage(
source: ImageSource.camera, maxWidth: 10.0, imageQuality: 70);
await picker.getImage(
source: ImageSource.camera, maxHeight: 10.0, imageQuality: 70);
await picker.getImage(
source: ImageSource.camera,
maxWidth: 10.0,
maxHeight: 20.0,
imageQuality: 70);
verifyInOrder(<Object>[
mockPlatform.pickImage(source: ImageSource.camera),
mockPlatform.pickImage(source: ImageSource.camera, maxWidth: 10.0),
mockPlatform.pickImage(source: ImageSource.camera, maxHeight: 10.0),
mockPlatform.pickImage(
source: ImageSource.camera,
maxWidth: 10.0,
maxHeight: 20.0,
),
mockPlatform.pickImage(
source: ImageSource.camera,
maxWidth: 10.0,
imageQuality: 70,
),
mockPlatform.pickImage(
source: ImageSource.camera,
maxHeight: 10.0,
imageQuality: 70,
),
mockPlatform.pickImage(
source: ImageSource.camera,
maxWidth: 10.0,
maxHeight: 20.0,
imageQuality: 70,
),
]);
});
test('handles a null image file response gracefully', () async {
final ImagePicker picker = ImagePicker();
expect(await picker.getImage(source: ImageSource.gallery), isNull);
expect(await picker.getImage(source: ImageSource.camera), isNull);
});
test('camera position defaults to back', () async {
final ImagePicker picker = ImagePicker();
await picker.getImage(source: ImageSource.camera);
verify(mockPlatform.pickImage(source: ImageSource.camera));
});
test('camera position can set to front', () async {
final ImagePicker picker = ImagePicker();
await picker.getImage(
source: ImageSource.camera,
preferredCameraDevice: CameraDevice.front);
verify(mockPlatform.pickImage(
source: ImageSource.camera,
preferredCameraDevice: CameraDevice.front));
});
});
group('#pickVideo', () {
setUp(() {
when(mockPlatform.pickVideo(
source: anyNamed('source'),
preferredCameraDevice: anyNamed('preferredCameraDevice'),
maxDuration: anyNamed('maxDuration')))
.thenAnswer((Invocation _) async => null);
});
test('passes the image source argument correctly', () async {
final ImagePicker picker = ImagePicker();
await picker.getVideo(source: ImageSource.camera);
await picker.getVideo(source: ImageSource.gallery);
verifyInOrder(<Object>[
mockPlatform.pickVideo(source: ImageSource.camera),
mockPlatform.pickVideo(source: ImageSource.gallery),
]);
});
test('passes the duration argument correctly', () async {
final ImagePicker picker = ImagePicker();
await picker.getVideo(source: ImageSource.camera);
await picker.getVideo(
source: ImageSource.camera,
maxDuration: const Duration(seconds: 10));
verifyInOrder(<Object>[
mockPlatform.pickVideo(source: ImageSource.camera),
mockPlatform.pickVideo(
source: ImageSource.camera,
maxDuration: const Duration(seconds: 10),
),
]);
});
test('handles a null video file response gracefully', () async {
final ImagePicker picker = ImagePicker();
expect(await picker.getVideo(source: ImageSource.gallery), isNull);
expect(await picker.getVideo(source: ImageSource.camera), isNull);
});
test('camera position defaults to back', () async {
final ImagePicker picker = ImagePicker();
await picker.getVideo(source: ImageSource.camera);
verify(mockPlatform.pickVideo(source: ImageSource.camera));
});
test('camera position can set to front', () async {
final ImagePicker picker = ImagePicker();
await picker.getVideo(
source: ImageSource.camera,
preferredCameraDevice: CameraDevice.front);
verify(mockPlatform.pickVideo(
source: ImageSource.camera,
preferredCameraDevice: CameraDevice.front));
});
});
group('#retrieveLostData', () {
test('retrieveLostData get success response', () async {
final ImagePicker picker = ImagePicker();
when(mockPlatform.retrieveLostData()).thenAnswer(
(Invocation _) async => LostData(
file: PickedFile('/example/path'), type: RetrieveType.image));
final LostData response = await picker.getLostData();
expect(response.type, RetrieveType.image);
expect(response.file!.path, '/example/path');
});
test('retrieveLostData get error response', () async {
final ImagePicker picker = ImagePicker();
when(mockPlatform.retrieveLostData()).thenAnswer(
(Invocation _) async => LostData(
exception: PlatformException(
code: 'test_error_code', message: 'test_error_message'),
type: RetrieveType.video));
final LostData response = await picker.getLostData();
expect(response.type, RetrieveType.video);
expect(response.exception!.code, 'test_error_code');
expect(response.exception!.message, 'test_error_message');
});
});
});
group('Multi images', () {
setUp(() {
when(mockPlatform.pickMultiImage(
maxWidth: anyNamed('maxWidth'),
maxHeight: anyNamed('maxHeight'),
imageQuality: anyNamed('imageQuality')))
.thenAnswer((Invocation _) async => null);
});
group('#pickMultiImage', () {
test('passes the width and height arguments correctly', () async {
final ImagePicker picker = ImagePicker();
await picker.getMultiImage();
await picker.getMultiImage(
maxWidth: 10.0,
);
await picker.getMultiImage(
maxHeight: 10.0,
);
await picker.getMultiImage(
maxWidth: 10.0,
maxHeight: 20.0,
);
await picker.getMultiImage(
maxWidth: 10.0,
imageQuality: 70,
);
await picker.getMultiImage(
maxHeight: 10.0,
imageQuality: 70,
);
await picker.getMultiImage(
maxWidth: 10.0, maxHeight: 20.0, imageQuality: 70);
verifyInOrder(<Object>[
mockPlatform.pickMultiImage(),
mockPlatform.pickMultiImage(maxWidth: 10.0),
mockPlatform.pickMultiImage(maxHeight: 10.0),
mockPlatform.pickMultiImage(maxWidth: 10.0, maxHeight: 20.0),
mockPlatform.pickMultiImage(maxWidth: 10.0, imageQuality: 70),
mockPlatform.pickMultiImage(maxHeight: 10.0, imageQuality: 70),
mockPlatform.pickMultiImage(
maxWidth: 10.0,
maxHeight: 20.0,
imageQuality: 70,
),
]);
});
test('handles a null image file response gracefully', () async {
final ImagePicker picker = ImagePicker();
expect(await picker.getMultiImage(), isNull);
expect(await picker.getMultiImage(), isNull);
});
});
});
});
}
| plugins/packages/image_picker/image_picker/test/image_picker_deprecated_test.dart/0 | {
"file_path": "plugins/packages/image_picker/image_picker/test/image_picker_deprecated_test.dart",
"repo_id": "plugins",
"token_count": 4651
} | 1,215 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.imagepicker;
import android.Manifest;
import android.content.Context;
import android.content.pm.PackageInfo;
import android.content.pm.PackageManager;
import android.os.Build;
import java.util.Arrays;
final class ImagePickerUtils {
/** returns true, if permission present in manifest, otherwise false */
private static boolean isPermissionPresentInManifest(Context context, String permissionName) {
try {
PackageManager packageManager = context.getPackageManager();
PackageInfo packageInfo =
packageManager.getPackageInfo(context.getPackageName(), PackageManager.GET_PERMISSIONS);
String[] requestedPermissions = packageInfo.requestedPermissions;
return Arrays.asList(requestedPermissions).contains(permissionName);
} catch (PackageManager.NameNotFoundException e) {
e.printStackTrace();
return false;
}
}
/**
* Camera permission need request if it present in manifest, because for M or great for take Photo
* ar Video by intent need it permission, even if the camera permission is not used.
*
* <p>Camera permission may be used in another package, as example flutter_barcode_reader.
* https://github.com/flutter/flutter/issues/29837
*
* @return returns true, if need request camera permission, otherwise false
*/
static boolean needRequestCameraPermission(Context context) {
boolean greatOrEqualM = Build.VERSION.SDK_INT >= Build.VERSION_CODES.M;
return greatOrEqualM && isPermissionPresentInManifest(context, Manifest.permission.CAMERA);
}
}
| plugins/packages/image_picker/image_picker_android/android/src/main/java/io/flutter/plugins/imagepicker/ImagePickerUtils.java/0 | {
"file_path": "plugins/packages/image_picker/image_picker_android/android/src/main/java/io/flutter/plugins/imagepicker/ImagePickerUtils.java",
"repo_id": "plugins",
"token_count": 516
} | 1,216 |
name: image_picker_for_web_integration_tests
publish_to: none
environment:
sdk: ">=2.12.0 <3.0.0"
flutter: ">=3.0.0"
dependencies:
flutter:
sdk: flutter
image_picker_for_web:
path: ../
image_picker_platform_interface: ^2.2.0
dev_dependencies:
flutter_driver:
sdk: flutter
flutter_test:
sdk: flutter
integration_test:
sdk: flutter
js: ^0.6.3
| plugins/packages/image_picker/image_picker_for_web/example/pubspec.yaml/0 | {
"file_path": "plugins/packages/image_picker/image_picker_for_web/example/pubspec.yaml",
"repo_id": "plugins",
"token_count": 180
} | 1,217 |
## 0.1.0+4
* Updates example code for `use_build_context_synchronously` lint.
* Updates minimum Flutter version to 3.0.
## 0.1.0+3
* Changes XTypeGroup initialization from final to const.
* Updates minimum Flutter version to 2.10.
## 0.1.0+2
* Minor fixes for new analysis options.
## 0.1.0+1
* Removes unnecessary imports.
* Fixes library_private_types_in_public_api, sort_child_properties_last and use_key_in_widget_constructors
lint warnings.
## 0.1.0
* Initial Windows support.
| plugins/packages/image_picker/image_picker_windows/CHANGELOG.md/0 | {
"file_path": "plugins/packages/image_picker/image_picker_windows/CHANGELOG.md",
"repo_id": "plugins",
"token_count": 169
} | 1,218 |
name: in_app_purchase
description: A Flutter plugin for in-app purchases. Exposes APIs for making in-app purchases through the App Store and Google Play.
repository: https://github.com/flutter/plugins/tree/main/packages/in_app_purchase/in_app_purchase
issue_tracker: https://github.com/flutter/flutter/issues?q=is%3Aissue+is%3Aopen+label%3A%22p%3A+in_app_purchase%22
version: 3.1.4
environment:
sdk: ">=2.12.0 <3.0.0"
flutter: ">=3.0.0"
flutter:
plugin:
platforms:
android:
default_package: in_app_purchase_android
ios:
default_package: in_app_purchase_storekit
macos:
default_package: in_app_purchase_storekit
dependencies:
flutter:
sdk: flutter
in_app_purchase_android: ^0.2.3
in_app_purchase_platform_interface: ^1.0.0
in_app_purchase_storekit: ^0.3.4
dev_dependencies:
flutter_driver:
sdk: flutter
flutter_test:
sdk: flutter
integration_test:
sdk: flutter
plugin_platform_interface: ^2.0.0
test: ^1.16.0
screenshots:
- description: 'Example of in-app purchase on ios'
path: doc/iap_ios.gif
- description: 'Example of in-app purchase on android'
path: doc/iap_android.gif
| plugins/packages/in_app_purchase/in_app_purchase/pubspec.yaml/0 | {
"file_path": "plugins/packages/in_app_purchase/in_app_purchase/pubspec.yaml",
"repo_id": "plugins",
"token_count": 491
} | 1,219 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/foundation.dart';
import 'package:flutter/services.dart';
import 'package:json_annotation/json_annotation.dart';
import '../../billing_client_wrappers.dart';
import '../channel.dart';
part 'billing_client_wrapper.g.dart';
/// Method identifier for the OnPurchaseUpdated method channel method.
@visibleForTesting
const String kOnPurchasesUpdated =
'PurchasesUpdatedListener#onPurchasesUpdated(int, List<Purchase>)';
const String _kOnBillingServiceDisconnected =
'BillingClientStateListener#onBillingServiceDisconnected()';
/// Callback triggered by Play in response to purchase activity.
///
/// This callback is triggered in response to all purchase activity while an
/// instance of `BillingClient` is active. This includes purchases initiated by
/// the app ([BillingClient.launchBillingFlow]) as well as purchases made in
/// Play itself while this app is open.
///
/// This does not provide any hooks for purchases made in the past. See
/// [BillingClient.queryPurchases] and [BillingClient.queryPurchaseHistory].
///
/// All purchase information should also be verified manually, with your server
/// if at all possible. See ["Verify a
/// purchase"](https://developer.android.com/google/play/billing/billing_library_overview#Verify).
///
/// Wraps a
/// [`PurchasesUpdatedListener`](https://developer.android.com/reference/com/android/billingclient/api/PurchasesUpdatedListener.html).
typedef PurchasesUpdatedListener = void Function(
PurchasesResultWrapper purchasesResult);
/// This class can be used directly instead of [InAppPurchaseConnection] to call
/// Play-specific billing APIs.
///
/// Wraps a
/// [`com.android.billingclient.api.BillingClient`](https://developer.android.com/reference/com/android/billingclient/api/BillingClient)
/// instance.
///
///
/// In general this API conforms to the Java
/// `com.android.billingclient.api.BillingClient` API as much as possible, with
/// some minor changes to account for language differences. Callbacks have been
/// converted to futures where appropriate.
class BillingClient {
/// Creates a billing client.
BillingClient(PurchasesUpdatedListener onPurchasesUpdated) {
channel.setMethodCallHandler(callHandler);
_callbacks[kOnPurchasesUpdated] = <PurchasesUpdatedListener>[
onPurchasesUpdated
];
}
// Occasionally methods in the native layer require a Dart callback to be
// triggered in response to a Java callback. For example,
// [startConnection] registers an [OnBillingServiceDisconnected] callback.
// This list of names to callbacks is used to trigger Dart callbacks in
// response to those Java callbacks. Dart sends the Java layer a handle to the
// matching callback here to remember, and then once its twin is triggered it
// sends the handle back over the platform channel. We then access that handle
// in this array and call it in Dart code. See also [_callHandler].
final Map<String, List<Function>> _callbacks = <String, List<Function>>{};
/// Calls
/// [`BillingClient#isReady()`](https://developer.android.com/reference/com/android/billingclient/api/BillingClient.html#isReady())
/// to get the ready status of the BillingClient instance.
Future<bool> isReady() async {
final bool? ready =
await channel.invokeMethod<bool>('BillingClient#isReady()');
return ready ?? false;
}
/// Enable the [BillingClientWrapper] to handle pending purchases.
///
/// **Deprecation warning:** it is no longer required to call
/// [enablePendingPurchases] when initializing your application.
@Deprecated(
'The requirement to call `enablePendingPurchases()` has become obsolete '
"since Google Play no longer accepts app submissions that don't support "
'pending purchases.')
void enablePendingPurchases() {
// No-op, until it is time to completely remove this method from the API.
}
/// Calls
/// [`BillingClient#startConnection(BillingClientStateListener)`](https://developer.android.com/reference/com/android/billingclient/api/BillingClient.html#startconnection)
/// to create and connect a `BillingClient` instance.
///
/// [onBillingServiceConnected] has been converted from a callback parameter
/// to the Future result returned by this function. This returns the
/// `BillingClient.BillingResultWrapper` describing the connection result.
///
/// This triggers the creation of a new `BillingClient` instance in Java if
/// one doesn't already exist.
Future<BillingResultWrapper> startConnection(
{required OnBillingServiceDisconnected
onBillingServiceDisconnected}) async {
final List<Function> disconnectCallbacks =
_callbacks[_kOnBillingServiceDisconnected] ??= <Function>[];
disconnectCallbacks.add(onBillingServiceDisconnected);
return BillingResultWrapper.fromJson((await channel
.invokeMapMethod<String, dynamic>(
'BillingClient#startConnection(BillingClientStateListener)',
<String, dynamic>{
'handle': disconnectCallbacks.length - 1,
})) ??
<String, dynamic>{});
}
/// Calls
/// [`BillingClient#endConnection(BillingClientStateListener)`](https://developer.android.com/reference/com/android/billingclient/api/BillingClient.html#endconnect
/// to disconnect a `BillingClient` instance.
///
/// Will trigger the [OnBillingServiceDisconnected] callback passed to [startConnection].
///
/// This triggers the destruction of the `BillingClient` instance in Java.
Future<void> endConnection() async {
return channel.invokeMethod<void>('BillingClient#endConnection()');
}
/// Returns a list of [SkuDetailsWrapper]s that have [SkuDetailsWrapper.sku]
/// in `skusList`, and [SkuDetailsWrapper.type] matching `skuType`.
///
/// Calls through to [`BillingClient#querySkuDetailsAsync(SkuDetailsParams,
/// SkuDetailsResponseListener)`](https://developer.android.com/reference/com/android/billingclient/api/BillingClient#querySkuDetailsAsync(com.android.billingclient.api.SkuDetailsParams,%20com.android.billingclient.api.SkuDetailsResponseListener))
/// Instead of taking a callback parameter, it returns a Future
/// [SkuDetailsResponseWrapper]. It also takes the values of
/// `SkuDetailsParams` as direct arguments instead of requiring it constructed
/// and passed in as a class.
Future<SkuDetailsResponseWrapper> querySkuDetails(
{required SkuType skuType, required List<String> skusList}) async {
final Map<String, dynamic> arguments = <String, dynamic>{
'skuType': const SkuTypeConverter().toJson(skuType),
'skusList': skusList
};
return SkuDetailsResponseWrapper.fromJson((await channel.invokeMapMethod<
String, dynamic>(
'BillingClient#querySkuDetailsAsync(SkuDetailsParams, SkuDetailsResponseListener)',
arguments)) ??
<String, dynamic>{});
}
/// Attempt to launch the Play Billing Flow for a given [skuDetails].
///
/// The [skuDetails] needs to have already been fetched in a [querySkuDetails]
/// call. The [accountId] is an optional hashed string associated with the user
/// that's unique to your app. It's used by Google to detect unusual behavior.
/// Do not pass in a cleartext [accountId], and do not use this field to store any Personally Identifiable Information (PII)
/// such as emails in cleartext. Attempting to store PII in this field will result in purchases being blocked.
/// Google Play recommends that you use either encryption or a one-way hash to generate an obfuscated identifier to send to Google Play.
///
/// Specifies an optional [obfuscatedProfileId] that is uniquely associated with the user's profile in your app.
/// Some applications allow users to have multiple profiles within a single account. Use this method to send the user's profile identifier to Google.
/// Setting this field requests the user's obfuscated account id.
///
/// Calling this attemps to show the Google Play purchase UI. The user is free
/// to complete the transaction there.
///
/// This method returns a [BillingResultWrapper] representing the initial attempt
/// to show the Google Play billing flow. Actual purchase updates are
/// delivered via the [PurchasesUpdatedListener].
///
/// This method calls through to
/// [`BillingClient#launchBillingFlow`](https://developer.android.com/reference/com/android/billingclient/api/BillingClient#launchbillingflow).
/// It constructs a
/// [`BillingFlowParams`](https://developer.android.com/reference/com/android/billingclient/api/BillingFlowParams)
/// instance by [setting the given skuDetails](https://developer.android.com/reference/com/android/billingclient/api/BillingFlowParams.Builder.html#setskudetails),
/// [the given accountId](https://developer.android.com/reference/com/android/billingclient/api/BillingFlowParams.Builder#setObfuscatedAccountId(java.lang.String))
/// and the [obfuscatedProfileId] (https://developer.android.com/reference/com/android/billingclient/api/BillingFlowParams.Builder#setobfuscatedprofileid).
///
/// When this method is called to purchase a subscription, an optional `oldSku`
/// can be passed in. This will tell Google Play that rather than purchasing a new subscription,
/// the user needs to upgrade/downgrade the existing subscription.
/// The [oldSku](https://developer.android.com/reference/com/android/billingclient/api/BillingFlowParams.Builder#setoldsku) and [purchaseToken] are the SKU id and purchase token that the user is upgrading or downgrading from.
/// [purchaseToken] must not be `null` if [oldSku] is not `null`.
/// The [prorationMode](https://developer.android.com/reference/com/android/billingclient/api/BillingFlowParams.Builder#setreplaceskusprorationmode) is the mode of proration during subscription upgrade/downgrade.
/// This value will only be effective if the `oldSku` is also set.
Future<BillingResultWrapper> launchBillingFlow(
{required String sku,
String? accountId,
String? obfuscatedProfileId,
String? oldSku,
String? purchaseToken,
ProrationMode? prorationMode}) async {
assert(sku != null);
assert((oldSku == null) == (purchaseToken == null),
'oldSku and purchaseToken must both be set, or both be null.');
final Map<String, dynamic> arguments = <String, dynamic>{
'sku': sku,
'accountId': accountId,
'obfuscatedProfileId': obfuscatedProfileId,
'oldSku': oldSku,
'purchaseToken': purchaseToken,
'prorationMode': const ProrationModeConverter().toJson(prorationMode ??
ProrationMode.unknownSubscriptionUpgradeDowngradePolicy)
};
return BillingResultWrapper.fromJson(
(await channel.invokeMapMethod<String, dynamic>(
'BillingClient#launchBillingFlow(Activity, BillingFlowParams)',
arguments)) ??
<String, dynamic>{});
}
/// Fetches recent purchases for the given [SkuType].
///
/// Unlike [queryPurchaseHistory], This does not make a network request and
/// does not return items that are no longer owned.
///
/// All purchase information should also be verified manually, with your
/// server if at all possible. See ["Verify a
/// purchase"](https://developer.android.com/google/play/billing/billing_library_overview#Verify).
///
/// This wraps [`BillingClient#queryPurchases(String
/// skutype)`](https://developer.android.com/reference/com/android/billingclient/api/BillingClient#querypurchases).
Future<PurchasesResultWrapper> queryPurchases(SkuType skuType) async {
assert(skuType != null);
return PurchasesResultWrapper.fromJson((await channel
.invokeMapMethod<String, dynamic>(
'BillingClient#queryPurchases(String)', <String, dynamic>{
'skuType': const SkuTypeConverter().toJson(skuType)
})) ??
<String, dynamic>{});
}
/// Fetches purchase history for the given [SkuType].
///
/// Unlike [queryPurchases], this makes a network request via Play and returns
/// the most recent purchase for each [SkuDetailsWrapper] of the given
/// [SkuType] even if the item is no longer owned.
///
/// All purchase information should also be verified manually, with your
/// server if at all possible. See ["Verify a
/// purchase"](https://developer.android.com/google/play/billing/billing_library_overview#Verify).
///
/// This wraps [`BillingClient#queryPurchaseHistoryAsync(String skuType,
/// PurchaseHistoryResponseListener
/// listener)`](https://developer.android.com/reference/com/android/billingclient/api/BillingClient#querypurchasehistoryasync).
Future<PurchasesHistoryResult> queryPurchaseHistory(SkuType skuType) async {
assert(skuType != null);
return PurchasesHistoryResult.fromJson((await channel.invokeMapMethod<
String, dynamic>(
'BillingClient#queryPurchaseHistoryAsync(String, PurchaseHistoryResponseListener)',
<String, dynamic>{
'skuType': const SkuTypeConverter().toJson(skuType)
})) ??
<String, dynamic>{});
}
/// Consumes a given in-app product.
///
/// Consuming can only be done on an item that's owned, and as a result of consumption, the user will no longer own it.
/// Consumption is done asynchronously. The method returns a Future containing a [BillingResultWrapper].
///
/// This wraps [`BillingClient#consumeAsync(String, ConsumeResponseListener)`](https://developer.android.com/reference/com/android/billingclient/api/BillingClient.html#consumeAsync(java.lang.String,%20com.android.billingclient.api.ConsumeResponseListener))
Future<BillingResultWrapper> consumeAsync(String purchaseToken) async {
assert(purchaseToken != null);
return BillingResultWrapper.fromJson((await channel
.invokeMapMethod<String, dynamic>(
'BillingClient#consumeAsync(String, ConsumeResponseListener)',
<String, dynamic>{
'purchaseToken': purchaseToken,
})) ??
<String, dynamic>{});
}
/// Acknowledge an in-app purchase.
///
/// The developer must acknowledge all in-app purchases after they have been granted to the user.
/// If this doesn't happen within three days of the purchase, the purchase will be refunded.
///
/// Consumables are already implicitly acknowledged by calls to [consumeAsync] and
/// do not need to be explicitly acknowledged by using this method.
/// However this method can be called for them in order to explicitly acknowledge them if desired.
///
/// Be sure to only acknowledge a purchase after it has been granted to the user.
/// [PurchaseWrapper.purchaseState] should be [PurchaseStateWrapper.purchased] and
/// the purchase should be validated. See [Verify a purchase](https://developer.android.com/google/play/billing/billing_library_overview#Verify) on verifying purchases.
///
/// Please refer to [acknowledge](https://developer.android.com/google/play/billing/billing_library_overview#acknowledge) for more
/// details.
///
/// This wraps [`BillingClient#acknowledgePurchase(String, AcknowledgePurchaseResponseListener)`](https://developer.android.com/reference/com/android/billingclient/api/BillingClient.html#acknowledgePurchase(com.android.billingclient.api.AcknowledgePurchaseParams,%20com.android.billingclient.api.AcknowledgePurchaseResponseListener))
Future<BillingResultWrapper> acknowledgePurchase(String purchaseToken) async {
assert(purchaseToken != null);
return BillingResultWrapper.fromJson((await channel.invokeMapMethod<String,
dynamic>(
'BillingClient#(AcknowledgePurchaseParams params, (AcknowledgePurchaseParams, AcknowledgePurchaseResponseListener)',
<String, dynamic>{
'purchaseToken': purchaseToken,
})) ??
<String, dynamic>{});
}
/// Checks if the specified feature or capability is supported by the Play Store.
/// Call this to check if a [BillingClientFeature] is supported by the device.
Future<bool> isFeatureSupported(BillingClientFeature feature) async {
final bool? result = await channel.invokeMethod<bool>(
'BillingClient#isFeatureSupported(String)', <String, dynamic>{
'feature': const BillingClientFeatureConverter().toJson(feature),
});
return result ?? false;
}
/// Initiates a flow to confirm the change of price for an item subscribed by the user.
///
/// When the price of a user subscribed item has changed, launch this flow to take users to
/// a screen with price change information. User can confirm the new price or cancel the flow.
///
/// The skuDetails needs to have already been fetched in a [querySkuDetails]
/// call.
Future<BillingResultWrapper> launchPriceChangeConfirmationFlow(
{required String sku}) async {
assert(sku != null);
final Map<String, dynamic> arguments = <String, dynamic>{
'sku': sku,
};
return BillingResultWrapper.fromJson((await channel.invokeMapMethod<String,
dynamic>(
'BillingClient#launchPriceChangeConfirmationFlow (Activity, PriceChangeFlowParams, PriceChangeConfirmationListener)',
arguments)) ??
<String, dynamic>{});
}
/// The method call handler for [channel].
@visibleForTesting
Future<void> callHandler(MethodCall call) async {
switch (call.method) {
case kOnPurchasesUpdated:
// The purchases updated listener is a singleton.
assert(_callbacks[kOnPurchasesUpdated]!.length == 1);
final PurchasesUpdatedListener listener =
_callbacks[kOnPurchasesUpdated]!.first as PurchasesUpdatedListener;
listener(PurchasesResultWrapper.fromJson(
(call.arguments as Map<dynamic, dynamic>).cast<String, dynamic>()));
break;
case _kOnBillingServiceDisconnected:
final int handle =
(call.arguments as Map<Object?, Object?>)['handle']! as int;
final List<OnBillingServiceDisconnected> onDisconnected =
_callbacks[_kOnBillingServiceDisconnected]!
.cast<OnBillingServiceDisconnected>();
onDisconnected[handle]();
break;
}
}
}
/// Callback triggered when the [BillingClientWrapper] is disconnected.
///
/// Wraps
/// [`com.android.billingclient.api.BillingClientStateListener.onServiceDisconnected()`](https://developer.android.com/reference/com/android/billingclient/api/BillingClientStateListener.html#onBillingServiceDisconnected())
/// to call back on `BillingClient` disconnect.
typedef OnBillingServiceDisconnected = void Function();
/// Possible `BillingClient` response statuses.
///
/// Wraps
/// [`BillingClient.BillingResponse`](https://developer.android.com/reference/com/android/billingclient/api/BillingClient.BillingResponse).
/// See the `BillingResponse` docs for more explanation of the different
/// constants.
@JsonEnum(alwaysCreate: true)
enum BillingResponse {
// WARNING: Changes to this class need to be reflected in our generated code.
// Run `flutter packages pub run build_runner watch` to rebuild and watch for
// further changes.
/// The request has reached the maximum timeout before Google Play responds.
@JsonValue(-3)
serviceTimeout,
/// The requested feature is not supported by Play Store on the current device.
@JsonValue(-2)
featureNotSupported,
/// The play Store service is not connected now - potentially transient state.
@JsonValue(-1)
serviceDisconnected,
/// Success.
@JsonValue(0)
ok,
/// The user pressed back or canceled a dialog.
@JsonValue(1)
userCanceled,
/// The network connection is down.
@JsonValue(2)
serviceUnavailable,
/// The billing API version is not supported for the type requested.
@JsonValue(3)
billingUnavailable,
/// The requested product is not available for purchase.
@JsonValue(4)
itemUnavailable,
/// Invalid arguments provided to the API.
@JsonValue(5)
developerError,
/// Fatal error during the API action.
@JsonValue(6)
error,
/// Failure to purchase since item is already owned.
@JsonValue(7)
itemAlreadyOwned,
/// Failure to consume since item is not owned.
@JsonValue(8)
itemNotOwned,
}
/// Serializer for [BillingResponse].
///
/// Use these in `@JsonSerializable()` classes by annotating them with
/// `@BillingResponseConverter()`.
class BillingResponseConverter implements JsonConverter<BillingResponse, int?> {
/// Default const constructor.
const BillingResponseConverter();
@override
BillingResponse fromJson(int? json) {
if (json == null) {
return BillingResponse.error;
}
return $enumDecode(_$BillingResponseEnumMap, json);
}
@override
int toJson(BillingResponse object) => _$BillingResponseEnumMap[object]!;
}
/// Enum representing potential [SkuDetailsWrapper.type]s.
///
/// Wraps
/// [`BillingClient.SkuType`](https://developer.android.com/reference/com/android/billingclient/api/BillingClient.SkuType)
/// See the linked documentation for an explanation of the different constants.
@JsonEnum(alwaysCreate: true)
enum SkuType {
// WARNING: Changes to this class need to be reflected in our generated code.
// Run `flutter packages pub run build_runner watch` to rebuild and watch for
// further changes.
/// A one time product. Acquired in a single transaction.
@JsonValue('inapp')
inapp,
/// A product requiring a recurring charge over time.
@JsonValue('subs')
subs,
}
/// Serializer for [SkuType].
///
/// Use these in `@JsonSerializable()` classes by annotating them with
/// `@SkuTypeConverter()`.
class SkuTypeConverter implements JsonConverter<SkuType, String?> {
/// Default const constructor.
const SkuTypeConverter();
@override
SkuType fromJson(String? json) {
if (json == null) {
return SkuType.inapp;
}
return $enumDecode(_$SkuTypeEnumMap, json);
}
@override
String toJson(SkuType object) => _$SkuTypeEnumMap[object]!;
}
/// Enum representing the proration mode.
///
/// When upgrading or downgrading a subscription, set this mode to provide details
/// about the proration that will be applied when the subscription changes.
///
/// Wraps [`BillingFlowParams.ProrationMode`](https://developer.android.com/reference/com/android/billingclient/api/BillingFlowParams.ProrationMode)
/// See the linked documentation for an explanation of the different constants.
@JsonEnum(alwaysCreate: true)
enum ProrationMode {
// WARNING: Changes to this class need to be reflected in our generated code.
// Run `flutter packages pub run build_runner watch` to rebuild and watch for
// further changes.
/// Unknown upgrade or downgrade policy.
@JsonValue(0)
unknownSubscriptionUpgradeDowngradePolicy,
/// Replacement takes effect immediately, and the remaining time will be prorated
/// and credited to the user.
///
/// This is the current default behavior.
@JsonValue(1)
immediateWithTimeProration,
/// Replacement takes effect immediately, and the billing cycle remains the same.
///
/// The price for the remaining period will be charged.
/// This option is only available for subscription upgrade.
@JsonValue(2)
immediateAndChargeProratedPrice,
/// Replacement takes effect immediately, and the new price will be charged on next
/// recurrence time.
///
/// The billing cycle stays the same.
@JsonValue(3)
immediateWithoutProration,
/// Replacement takes effect when the old plan expires, and the new price will
/// be charged at the same time.
@JsonValue(4)
deferred,
/// Replacement takes effect immediately, and the user is charged full price
/// of new plan and is given a full billing cycle of subscription, plus
/// remaining prorated time from the old plan.
@JsonValue(5)
immediateAndChargeFullPrice,
}
/// Serializer for [ProrationMode].
///
/// Use these in `@JsonSerializable()` classes by annotating them with
/// `@ProrationModeConverter()`.
class ProrationModeConverter implements JsonConverter<ProrationMode, int?> {
/// Default const constructor.
const ProrationModeConverter();
@override
ProrationMode fromJson(int? json) {
if (json == null) {
return ProrationMode.unknownSubscriptionUpgradeDowngradePolicy;
}
return $enumDecode(_$ProrationModeEnumMap, json);
}
@override
int toJson(ProrationMode object) => _$ProrationModeEnumMap[object]!;
}
/// Features/capabilities supported by [BillingClient.isFeatureSupported()](https://developer.android.com/reference/com/android/billingclient/api/BillingClient.FeatureType).
@JsonEnum(alwaysCreate: true)
enum BillingClientFeature {
// WARNING: Changes to this class need to be reflected in our generated code.
// Run `flutter packages pub run build_runner watch` to rebuild and watch for
// further changes.
// JsonValues need to match constant values defined in https://developer.android.com/reference/com/android/billingclient/api/BillingClient.FeatureType#summary
/// Purchase/query for in-app items on VR.
@JsonValue('inAppItemsOnVr')
inAppItemsOnVR,
/// Launch a price change confirmation flow.
@JsonValue('priceChangeConfirmation')
priceChangeConfirmation,
/// Purchase/query for subscriptions.
@JsonValue('subscriptions')
subscriptions,
/// Purchase/query for subscriptions on VR.
@JsonValue('subscriptionsOnVr')
subscriptionsOnVR,
/// Subscriptions update/replace.
@JsonValue('subscriptionsUpdate')
subscriptionsUpdate
}
/// Serializer for [BillingClientFeature].
///
/// Use these in `@JsonSerializable()` classes by annotating them with
/// `@BillingClientFeatureConverter()`.
class BillingClientFeatureConverter
implements JsonConverter<BillingClientFeature, String> {
/// Default const constructor.
const BillingClientFeatureConverter();
@override
BillingClientFeature fromJson(String json) {
return $enumDecode<BillingClientFeature, dynamic>(
_$BillingClientFeatureEnumMap.cast<BillingClientFeature, dynamic>(),
json);
}
@override
String toJson(BillingClientFeature object) =>
_$BillingClientFeatureEnumMap[object]!;
}
| plugins/packages/in_app_purchase/in_app_purchase_android/lib/src/billing_client_wrappers/billing_client_wrapper.dart/0 | {
"file_path": "plugins/packages/in_app_purchase/in_app_purchase_android/lib/src/billing_client_wrappers/billing_client_wrapper.dart",
"repo_id": "plugins",
"token_count": 8119
} | 1,220 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:in_app_purchase_android/billing_client_wrappers.dart';
import 'package:in_app_purchase_android/src/channel.dart';
import '../stub_in_app_purchase_platform.dart';
import 'purchase_wrapper_test.dart';
import 'sku_details_wrapper_test.dart';
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
final StubInAppPurchasePlatform stubPlatform = StubInAppPurchasePlatform();
late BillingClient billingClient;
setUpAll(() => _ambiguate(TestDefaultBinaryMessengerBinding.instance)!
.defaultBinaryMessenger
.setMockMethodCallHandler(channel, stubPlatform.fakeMethodCallHandler));
setUp(() {
billingClient = BillingClient((PurchasesResultWrapper _) {});
stubPlatform.reset();
});
group('isReady', () {
test('true', () async {
stubPlatform.addResponse(name: 'BillingClient#isReady()', value: true);
expect(await billingClient.isReady(), isTrue);
});
test('false', () async {
stubPlatform.addResponse(name: 'BillingClient#isReady()', value: false);
expect(await billingClient.isReady(), isFalse);
});
});
// Make sure that the enum values are supported and that the converter call
// does not fail
test('response states', () async {
const BillingResponseConverter converter = BillingResponseConverter();
converter.fromJson(-3);
converter.fromJson(-2);
converter.fromJson(-1);
converter.fromJson(0);
converter.fromJson(1);
converter.fromJson(2);
converter.fromJson(3);
converter.fromJson(4);
converter.fromJson(5);
converter.fromJson(6);
converter.fromJson(7);
converter.fromJson(8);
});
group('startConnection', () {
const String methodName =
'BillingClient#startConnection(BillingClientStateListener)';
test('returns BillingResultWrapper', () async {
const String debugMessage = 'dummy message';
const BillingResponse responseCode = BillingResponse.developerError;
stubPlatform.addResponse(
name: methodName,
value: <String, dynamic>{
'responseCode': const BillingResponseConverter().toJson(responseCode),
'debugMessage': debugMessage,
},
);
const BillingResultWrapper billingResult = BillingResultWrapper(
responseCode: responseCode, debugMessage: debugMessage);
expect(
await billingClient.startConnection(
onBillingServiceDisconnected: () {}),
equals(billingResult));
});
test('passes handle to onBillingServiceDisconnected', () async {
const String debugMessage = 'dummy message';
const BillingResponse responseCode = BillingResponse.developerError;
stubPlatform.addResponse(
name: methodName,
value: <String, dynamic>{
'responseCode': const BillingResponseConverter().toJson(responseCode),
'debugMessage': debugMessage,
},
);
await billingClient.startConnection(onBillingServiceDisconnected: () {});
final MethodCall call = stubPlatform.previousCallMatching(methodName);
expect(call.arguments, equals(<dynamic, dynamic>{'handle': 0}));
});
test('handles method channel returning null', () async {
stubPlatform.addResponse(
name: methodName,
);
expect(
await billingClient.startConnection(
onBillingServiceDisconnected: () {}),
equals(const BillingResultWrapper(
responseCode: BillingResponse.error,
debugMessage: kInvalidBillingResultErrorMessage)));
});
});
test('endConnection', () async {
const String endConnectionName = 'BillingClient#endConnection()';
expect(stubPlatform.countPreviousCalls(endConnectionName), equals(0));
stubPlatform.addResponse(name: endConnectionName);
await billingClient.endConnection();
expect(stubPlatform.countPreviousCalls(endConnectionName), equals(1));
});
group('querySkuDetails', () {
const String queryMethodName =
'BillingClient#querySkuDetailsAsync(SkuDetailsParams, SkuDetailsResponseListener)';
test('handles empty skuDetails', () async {
const String debugMessage = 'dummy message';
const BillingResponse responseCode = BillingResponse.developerError;
stubPlatform.addResponse(name: queryMethodName, value: <dynamic, dynamic>{
'billingResult': <String, dynamic>{
'responseCode': const BillingResponseConverter().toJson(responseCode),
'debugMessage': debugMessage,
},
'skuDetailsList': <Map<String, dynamic>>[]
});
final SkuDetailsResponseWrapper response = await billingClient
.querySkuDetails(
skuType: SkuType.inapp, skusList: <String>['invalid']);
const BillingResultWrapper billingResult = BillingResultWrapper(
responseCode: responseCode, debugMessage: debugMessage);
expect(response.billingResult, equals(billingResult));
expect(response.skuDetailsList, isEmpty);
});
test('returns SkuDetailsResponseWrapper', () async {
const String debugMessage = 'dummy message';
const BillingResponse responseCode = BillingResponse.ok;
stubPlatform.addResponse(name: queryMethodName, value: <String, dynamic>{
'billingResult': <String, dynamic>{
'responseCode': const BillingResponseConverter().toJson(responseCode),
'debugMessage': debugMessage,
},
'skuDetailsList': <Map<String, dynamic>>[buildSkuMap(dummySkuDetails)]
});
final SkuDetailsResponseWrapper response = await billingClient
.querySkuDetails(
skuType: SkuType.inapp, skusList: <String>['invalid']);
const BillingResultWrapper billingResult = BillingResultWrapper(
responseCode: responseCode, debugMessage: debugMessage);
expect(response.billingResult, equals(billingResult));
expect(response.skuDetailsList, contains(dummySkuDetails));
});
test('handles null method channel response', () async {
stubPlatform.addResponse(name: queryMethodName);
final SkuDetailsResponseWrapper response = await billingClient
.querySkuDetails(
skuType: SkuType.inapp, skusList: <String>['invalid']);
const BillingResultWrapper billingResult = BillingResultWrapper(
responseCode: BillingResponse.error,
debugMessage: kInvalidBillingResultErrorMessage);
expect(response.billingResult, equals(billingResult));
expect(response.skuDetailsList, isEmpty);
});
});
group('launchBillingFlow', () {
const String launchMethodName =
'BillingClient#launchBillingFlow(Activity, BillingFlowParams)';
test('serializes and deserializes data', () async {
const String debugMessage = 'dummy message';
const BillingResponse responseCode = BillingResponse.ok;
const BillingResultWrapper expectedBillingResult = BillingResultWrapper(
responseCode: responseCode, debugMessage: debugMessage);
stubPlatform.addResponse(
name: launchMethodName,
value: buildBillingResultMap(expectedBillingResult),
);
const SkuDetailsWrapper skuDetails = dummySkuDetails;
const String accountId = 'hashedAccountId';
const String profileId = 'hashedProfileId';
expect(
await billingClient.launchBillingFlow(
sku: skuDetails.sku,
accountId: accountId,
obfuscatedProfileId: profileId),
equals(expectedBillingResult));
final Map<dynamic, dynamic> arguments = stubPlatform
.previousCallMatching(launchMethodName)
.arguments as Map<dynamic, dynamic>;
expect(arguments['sku'], equals(skuDetails.sku));
expect(arguments['accountId'], equals(accountId));
expect(arguments['obfuscatedProfileId'], equals(profileId));
});
test(
'Change subscription throws assertion error `oldSku` and `purchaseToken` has different nullability',
() async {
const String debugMessage = 'dummy message';
const BillingResponse responseCode = BillingResponse.ok;
const BillingResultWrapper expectedBillingResult = BillingResultWrapper(
responseCode: responseCode, debugMessage: debugMessage);
stubPlatform.addResponse(
name: launchMethodName,
value: buildBillingResultMap(expectedBillingResult),
);
const SkuDetailsWrapper skuDetails = dummySkuDetails;
const String accountId = 'hashedAccountId';
const String profileId = 'hashedProfileId';
expect(
billingClient.launchBillingFlow(
sku: skuDetails.sku,
accountId: accountId,
obfuscatedProfileId: profileId,
oldSku: dummyOldPurchase.sku),
throwsAssertionError);
expect(
billingClient.launchBillingFlow(
sku: skuDetails.sku,
accountId: accountId,
obfuscatedProfileId: profileId,
purchaseToken: dummyOldPurchase.purchaseToken),
throwsAssertionError);
});
test(
'serializes and deserializes data on change subscription without proration',
() async {
const String debugMessage = 'dummy message';
const BillingResponse responseCode = BillingResponse.ok;
const BillingResultWrapper expectedBillingResult = BillingResultWrapper(
responseCode: responseCode, debugMessage: debugMessage);
stubPlatform.addResponse(
name: launchMethodName,
value: buildBillingResultMap(expectedBillingResult),
);
const SkuDetailsWrapper skuDetails = dummySkuDetails;
const String accountId = 'hashedAccountId';
const String profileId = 'hashedProfileId';
expect(
await billingClient.launchBillingFlow(
sku: skuDetails.sku,
accountId: accountId,
obfuscatedProfileId: profileId,
oldSku: dummyOldPurchase.sku,
purchaseToken: dummyOldPurchase.purchaseToken),
equals(expectedBillingResult));
final Map<dynamic, dynamic> arguments = stubPlatform
.previousCallMatching(launchMethodName)
.arguments as Map<dynamic, dynamic>;
expect(arguments['sku'], equals(skuDetails.sku));
expect(arguments['accountId'], equals(accountId));
expect(arguments['oldSku'], equals(dummyOldPurchase.sku));
expect(
arguments['purchaseToken'], equals(dummyOldPurchase.purchaseToken));
expect(arguments['obfuscatedProfileId'], equals(profileId));
});
test(
'serializes and deserializes data on change subscription with proration',
() async {
const String debugMessage = 'dummy message';
const BillingResponse responseCode = BillingResponse.ok;
const BillingResultWrapper expectedBillingResult = BillingResultWrapper(
responseCode: responseCode, debugMessage: debugMessage);
stubPlatform.addResponse(
name: launchMethodName,
value: buildBillingResultMap(expectedBillingResult),
);
const SkuDetailsWrapper skuDetails = dummySkuDetails;
const String accountId = 'hashedAccountId';
const String profileId = 'hashedProfileId';
const ProrationMode prorationMode =
ProrationMode.immediateAndChargeProratedPrice;
expect(
await billingClient.launchBillingFlow(
sku: skuDetails.sku,
accountId: accountId,
obfuscatedProfileId: profileId,
oldSku: dummyOldPurchase.sku,
prorationMode: prorationMode,
purchaseToken: dummyOldPurchase.purchaseToken),
equals(expectedBillingResult));
final Map<dynamic, dynamic> arguments = stubPlatform
.previousCallMatching(launchMethodName)
.arguments as Map<dynamic, dynamic>;
expect(arguments['sku'], equals(skuDetails.sku));
expect(arguments['accountId'], equals(accountId));
expect(arguments['oldSku'], equals(dummyOldPurchase.sku));
expect(arguments['obfuscatedProfileId'], equals(profileId));
expect(
arguments['purchaseToken'], equals(dummyOldPurchase.purchaseToken));
expect(arguments['prorationMode'],
const ProrationModeConverter().toJson(prorationMode));
});
test(
'serializes and deserializes data when using immediateAndChargeFullPrice',
() async {
const String debugMessage = 'dummy message';
const BillingResponse responseCode = BillingResponse.ok;
const BillingResultWrapper expectedBillingResult = BillingResultWrapper(
responseCode: responseCode, debugMessage: debugMessage);
stubPlatform.addResponse(
name: launchMethodName,
value: buildBillingResultMap(expectedBillingResult),
);
const SkuDetailsWrapper skuDetails = dummySkuDetails;
const String accountId = 'hashedAccountId';
const String profileId = 'hashedProfileId';
const ProrationMode prorationMode =
ProrationMode.immediateAndChargeFullPrice;
expect(
await billingClient.launchBillingFlow(
sku: skuDetails.sku,
accountId: accountId,
obfuscatedProfileId: profileId,
oldSku: dummyOldPurchase.sku,
prorationMode: prorationMode,
purchaseToken: dummyOldPurchase.purchaseToken),
equals(expectedBillingResult));
final Map<dynamic, dynamic> arguments = stubPlatform
.previousCallMatching(launchMethodName)
.arguments as Map<dynamic, dynamic>;
expect(arguments['sku'], equals(skuDetails.sku));
expect(arguments['accountId'], equals(accountId));
expect(arguments['oldSku'], equals(dummyOldPurchase.sku));
expect(arguments['obfuscatedProfileId'], equals(profileId));
expect(
arguments['purchaseToken'], equals(dummyOldPurchase.purchaseToken));
expect(arguments['prorationMode'],
const ProrationModeConverter().toJson(prorationMode));
});
test('handles null accountId', () async {
const String debugMessage = 'dummy message';
const BillingResponse responseCode = BillingResponse.ok;
const BillingResultWrapper expectedBillingResult = BillingResultWrapper(
responseCode: responseCode, debugMessage: debugMessage);
stubPlatform.addResponse(
name: launchMethodName,
value: buildBillingResultMap(expectedBillingResult),
);
const SkuDetailsWrapper skuDetails = dummySkuDetails;
expect(await billingClient.launchBillingFlow(sku: skuDetails.sku),
equals(expectedBillingResult));
final Map<dynamic, dynamic> arguments = stubPlatform
.previousCallMatching(launchMethodName)
.arguments as Map<dynamic, dynamic>;
expect(arguments['sku'], equals(skuDetails.sku));
expect(arguments['accountId'], isNull);
});
test('handles method channel returning null', () async {
stubPlatform.addResponse(
name: launchMethodName,
);
const SkuDetailsWrapper skuDetails = dummySkuDetails;
expect(
await billingClient.launchBillingFlow(sku: skuDetails.sku),
equals(const BillingResultWrapper(
responseCode: BillingResponse.error,
debugMessage: kInvalidBillingResultErrorMessage)));
});
});
group('queryPurchases', () {
const String queryPurchasesMethodName =
'BillingClient#queryPurchases(String)';
test('serializes and deserializes data', () async {
const BillingResponse expectedCode = BillingResponse.ok;
final List<PurchaseWrapper> expectedList = <PurchaseWrapper>[
dummyPurchase
];
const String debugMessage = 'dummy message';
const BillingResultWrapper expectedBillingResult = BillingResultWrapper(
responseCode: expectedCode, debugMessage: debugMessage);
stubPlatform
.addResponse(name: queryPurchasesMethodName, value: <String, dynamic>{
'billingResult': buildBillingResultMap(expectedBillingResult),
'responseCode': const BillingResponseConverter().toJson(expectedCode),
'purchasesList': expectedList
.map((PurchaseWrapper purchase) => buildPurchaseMap(purchase))
.toList(),
});
final PurchasesResultWrapper response =
await billingClient.queryPurchases(SkuType.inapp);
expect(response.billingResult, equals(expectedBillingResult));
expect(response.responseCode, equals(expectedCode));
expect(response.purchasesList, equals(expectedList));
});
test('handles empty purchases', () async {
const BillingResponse expectedCode = BillingResponse.userCanceled;
const String debugMessage = 'dummy message';
const BillingResultWrapper expectedBillingResult = BillingResultWrapper(
responseCode: expectedCode, debugMessage: debugMessage);
stubPlatform
.addResponse(name: queryPurchasesMethodName, value: <String, dynamic>{
'billingResult': buildBillingResultMap(expectedBillingResult),
'responseCode': const BillingResponseConverter().toJson(expectedCode),
'purchasesList': <dynamic>[],
});
final PurchasesResultWrapper response =
await billingClient.queryPurchases(SkuType.inapp);
expect(response.billingResult, equals(expectedBillingResult));
expect(response.responseCode, equals(expectedCode));
expect(response.purchasesList, isEmpty);
});
test('handles method channel returning null', () async {
stubPlatform.addResponse(
name: queryPurchasesMethodName,
);
final PurchasesResultWrapper response =
await billingClient.queryPurchases(SkuType.inapp);
expect(
response.billingResult,
equals(const BillingResultWrapper(
responseCode: BillingResponse.error,
debugMessage: kInvalidBillingResultErrorMessage)));
expect(response.responseCode, BillingResponse.error);
expect(response.purchasesList, isEmpty);
});
});
group('queryPurchaseHistory', () {
const String queryPurchaseHistoryMethodName =
'BillingClient#queryPurchaseHistoryAsync(String, PurchaseHistoryResponseListener)';
test('serializes and deserializes data', () async {
const BillingResponse expectedCode = BillingResponse.ok;
final List<PurchaseHistoryRecordWrapper> expectedList =
<PurchaseHistoryRecordWrapper>[
dummyPurchaseHistoryRecord,
];
const String debugMessage = 'dummy message';
const BillingResultWrapper expectedBillingResult = BillingResultWrapper(
responseCode: expectedCode, debugMessage: debugMessage);
stubPlatform.addResponse(
name: queryPurchaseHistoryMethodName,
value: <String, dynamic>{
'billingResult': buildBillingResultMap(expectedBillingResult),
'purchaseHistoryRecordList': expectedList
.map((PurchaseHistoryRecordWrapper purchaseHistoryRecord) =>
buildPurchaseHistoryRecordMap(purchaseHistoryRecord))
.toList(),
});
final PurchasesHistoryResult response =
await billingClient.queryPurchaseHistory(SkuType.inapp);
expect(response.billingResult, equals(expectedBillingResult));
expect(response.purchaseHistoryRecordList, equals(expectedList));
});
test('handles empty purchases', () async {
const BillingResponse expectedCode = BillingResponse.userCanceled;
const String debugMessage = 'dummy message';
const BillingResultWrapper expectedBillingResult = BillingResultWrapper(
responseCode: expectedCode, debugMessage: debugMessage);
stubPlatform.addResponse(
name: queryPurchaseHistoryMethodName,
value: <dynamic, dynamic>{
'billingResult': buildBillingResultMap(expectedBillingResult),
'purchaseHistoryRecordList': <dynamic>[],
});
final PurchasesHistoryResult response =
await billingClient.queryPurchaseHistory(SkuType.inapp);
expect(response.billingResult, equals(expectedBillingResult));
expect(response.purchaseHistoryRecordList, isEmpty);
});
test('handles method channel returning null', () async {
stubPlatform.addResponse(
name: queryPurchaseHistoryMethodName,
);
final PurchasesHistoryResult response =
await billingClient.queryPurchaseHistory(SkuType.inapp);
expect(
response.billingResult,
equals(const BillingResultWrapper(
responseCode: BillingResponse.error,
debugMessage: kInvalidBillingResultErrorMessage)));
expect(response.purchaseHistoryRecordList, isEmpty);
});
});
group('consume purchases', () {
const String consumeMethodName =
'BillingClient#consumeAsync(String, ConsumeResponseListener)';
test('consume purchase async success', () async {
const BillingResponse expectedCode = BillingResponse.ok;
const String debugMessage = 'dummy message';
const BillingResultWrapper expectedBillingResult = BillingResultWrapper(
responseCode: expectedCode, debugMessage: debugMessage);
stubPlatform.addResponse(
name: consumeMethodName,
value: buildBillingResultMap(expectedBillingResult));
final BillingResultWrapper billingResult =
await billingClient.consumeAsync('dummy token');
expect(billingResult, equals(expectedBillingResult));
});
test('handles method channel returning null', () async {
stubPlatform.addResponse(
name: consumeMethodName,
);
final BillingResultWrapper billingResult =
await billingClient.consumeAsync('dummy token');
expect(
billingResult,
equals(const BillingResultWrapper(
responseCode: BillingResponse.error,
debugMessage: kInvalidBillingResultErrorMessage)));
});
});
group('acknowledge purchases', () {
const String acknowledgeMethodName =
'BillingClient#(AcknowledgePurchaseParams params, (AcknowledgePurchaseParams, AcknowledgePurchaseResponseListener)';
test('acknowledge purchase success', () async {
const BillingResponse expectedCode = BillingResponse.ok;
const String debugMessage = 'dummy message';
const BillingResultWrapper expectedBillingResult = BillingResultWrapper(
responseCode: expectedCode, debugMessage: debugMessage);
stubPlatform.addResponse(
name: acknowledgeMethodName,
value: buildBillingResultMap(expectedBillingResult));
final BillingResultWrapper billingResult =
await billingClient.acknowledgePurchase('dummy token');
expect(billingResult, equals(expectedBillingResult));
});
test('handles method channel returning null', () async {
stubPlatform.addResponse(
name: acknowledgeMethodName,
);
final BillingResultWrapper billingResult =
await billingClient.acknowledgePurchase('dummy token');
expect(
billingResult,
equals(const BillingResultWrapper(
responseCode: BillingResponse.error,
debugMessage: kInvalidBillingResultErrorMessage)));
});
});
group('isFeatureSupported', () {
const String isFeatureSupportedMethodName =
'BillingClient#isFeatureSupported(String)';
test('isFeatureSupported returns false', () async {
late Map<Object?, Object?> arguments;
stubPlatform.addResponse(
name: isFeatureSupportedMethodName,
value: false,
additionalStepBeforeReturn: (dynamic value) =>
arguments = value as Map<dynamic, dynamic>,
);
final bool isSupported = await billingClient
.isFeatureSupported(BillingClientFeature.subscriptions);
expect(isSupported, isFalse);
expect(arguments['feature'], equals('subscriptions'));
});
test('isFeatureSupported returns true', () async {
late Map<Object?, Object?> arguments;
stubPlatform.addResponse(
name: isFeatureSupportedMethodName,
value: true,
additionalStepBeforeReturn: (dynamic value) =>
arguments = value as Map<dynamic, dynamic>,
);
final bool isSupported = await billingClient
.isFeatureSupported(BillingClientFeature.subscriptions);
expect(isSupported, isTrue);
expect(arguments['feature'], equals('subscriptions'));
});
});
group('launchPriceChangeConfirmationFlow', () {
const String launchPriceChangeConfirmationFlowMethodName =
'BillingClient#launchPriceChangeConfirmationFlow (Activity, PriceChangeFlowParams, PriceChangeConfirmationListener)';
const BillingResultWrapper expectedBillingResultPriceChangeConfirmation =
BillingResultWrapper(
responseCode: BillingResponse.ok,
debugMessage: 'dummy message',
);
test('serializes and deserializes data', () async {
stubPlatform.addResponse(
name: launchPriceChangeConfirmationFlowMethodName,
value:
buildBillingResultMap(expectedBillingResultPriceChangeConfirmation),
);
expect(
await billingClient.launchPriceChangeConfirmationFlow(
sku: dummySkuDetails.sku,
),
equals(expectedBillingResultPriceChangeConfirmation),
);
});
test('passes sku to launchPriceChangeConfirmationFlow', () async {
stubPlatform.addResponse(
name: launchPriceChangeConfirmationFlowMethodName,
value:
buildBillingResultMap(expectedBillingResultPriceChangeConfirmation),
);
await billingClient.launchPriceChangeConfirmationFlow(
sku: dummySkuDetails.sku,
);
final MethodCall call = stubPlatform
.previousCallMatching(launchPriceChangeConfirmationFlowMethodName);
expect(call.arguments,
equals(<dynamic, dynamic>{'sku': dummySkuDetails.sku}));
});
});
}
/// This allows a value of type T or T? to be treated as a value of type T?.
///
/// We use this so that APIs that have become non-nullable can still be used
/// with `!` and `?` on the stable branch.
T? _ambiguate<T>(T? value) => value;
| plugins/packages/in_app_purchase/in_app_purchase_android/test/billing_client_wrappers/billing_client_wrapper_test.dart/0 | {
"file_path": "plugins/packages/in_app_purchase/in_app_purchase_android/test/billing_client_wrappers/billing_client_wrapper_test.dart",
"repo_id": "plugins",
"token_count": 9811
} | 1,221 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import '../in_app_purchase_platform_interface.dart';
// ignore: avoid_classes_with_only_static_members
/// The interface that platform implementations must implement when they want to
/// provide platform-specific in_app_purchase features.
///
/// Platforms that wants to introduce platform-specific public APIs should create
/// a class that either extend or implements [InAppPurchasePlatformAddition]. Then set
/// the [InAppPurchasePlatformAddition.instance] to an instance of that class.
///
/// All the APIs added by [InAppPurchasePlatformAddition] implementations will be accessed from
/// [InAppPurchasePlatformAdditionProvider.getPlatformAddition] by the client APPs.
/// To avoid clients directly calling [InAppPurchasePlatform] APIs,
/// an [InAppPurchasePlatformAddition] implementation should not be a type of [InAppPurchasePlatform].
abstract class InAppPurchasePlatformAddition {
static InAppPurchasePlatformAddition? _instance;
/// The instance containing the platform-specific in_app_purchase
/// functionality.
///
/// Returns `null` by default.
///
/// To implement additional functionality extend
/// [`InAppPurchasePlatformAddition`][3] with the platform-specific
/// functionality, and when the plugin is registered, set the
/// `InAppPurchasePlatformAddition.instance` with the new addition
/// implementation instance.
///
/// Example implementation might look like this:
/// ```dart
/// class InAppPurchaseMyPlatformAddition extends InAppPurchasePlatformAddition {
/// Future<void> myPlatformMethod() {}
/// }
/// ```
///
/// The following snippet shows how to register the `InAppPurchaseMyPlatformAddition`:
/// ```dart
/// class InAppPurchaseMyPlatformPlugin {
/// static void registerWith(Registrar registrar) {
/// // Register the platform-specific implementation of the idiomatic
/// // InAppPurchase API.
/// InAppPurchasePlatform.instance = InAppPurchaseMyPlatformPlugin();
///
/// // Register the [InAppPurchaseMyPlatformAddition] containing the
/// // platform-specific functionality.
/// InAppPurchasePlatformAddition.instance = InAppPurchaseMyPlatformAddition();
/// }
/// }
/// ```
static InAppPurchasePlatformAddition? get instance => _instance;
/// Sets the instance to a desired [InAppPurchasePlatformAddition] implementation.
///
/// The `instance` should not be a type of [InAppPurchasePlatform].
static set instance(InAppPurchasePlatformAddition? instance) {
assert(instance is! InAppPurchasePlatform);
_instance = instance;
}
}
| plugins/packages/in_app_purchase/in_app_purchase_platform_interface/lib/src/in_app_purchase_platform_addition.dart/0 | {
"file_path": "plugins/packages/in_app_purchase/in_app_purchase_platform_interface/lib/src/in_app_purchase_platform_addition.dart",
"repo_id": "plugins",
"token_count": 718
} | 1,222 |
#include "../../Flutter/Flutter-Debug.xcconfig"
| plugins/packages/in_app_purchase/in_app_purchase_storekit/example/macos/Runner/Configs/Debug.xcconfig/0 | {
"file_path": "plugins/packages/in_app_purchase/in_app_purchase_storekit/example/macos/Runner/Configs/Debug.xcconfig",
"repo_id": "plugins",
"token_count": 19
} | 1,223 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import '../../store_kit_wrappers.dart';
/// A wrapper around
/// [`SKPaymentQueueDelegate`](https://developer.apple.com/documentation/storekit/skpaymentqueuedelegate?language=objc).
///
/// The payment queue delegate can be implementated to provide information
/// needed to complete transactions.
///
/// The [SKPaymentQueueDelegateWrapper] is available on macOS and iOS 13+.
/// Usage with versions below iOS 13 and macOS are ignored.
abstract class SKPaymentQueueDelegateWrapper {
/// Called by the system to check whether the transaction should continue if
/// the device's App Store storefront has changed during a transaction.
///
/// - Return `true` if the transaction should continue within the updated
/// storefront (default behaviour).
/// - Return `false` if the transaction should be cancelled. In this case the
/// transaction will fail with the error [SKErrorStoreProductNotAvailable](https://developer.apple.com/documentation/storekit/skerrorcode/skerrorstoreproductnotavailable?language=objc).
///
/// See the documentation in StoreKit's [`[-SKPaymentQueueDelegate shouldContinueTransaction]`](https://developer.apple.com/documentation/storekit/skpaymentqueuedelegate/3242935-paymentqueue?language=objc).
bool shouldContinueTransaction(
SKPaymentTransactionWrapper transaction,
SKStorefrontWrapper storefront,
) =>
true;
/// Called by the system to check whether to immediately show the price
/// consent form.
///
/// The default return value is `true`. This will inform the system to display
/// the price consent sheet when the subscription price has been changed in
/// App Store Connect and the subscriber has not yet taken action. See the
/// documentation in StoreKit's [`[-SKPaymentQueueDelegate shouldShowPriceConsent:]`](https://developer.apple.com/documentation/storekit/skpaymentqueuedelegate/3521328-paymentqueueshouldshowpriceconse?language=objc).
bool shouldShowPriceConsent() => true;
}
| plugins/packages/in_app_purchase/in_app_purchase_storekit/lib/src/store_kit_wrappers/sk_payment_queue_delegate_wrapper.dart/0 | {
"file_path": "plugins/packages/in_app_purchase/in_app_purchase_storekit/lib/src/store_kit_wrappers/sk_payment_queue_delegate_wrapper.dart",
"repo_id": "plugins",
"token_count": 561
} | 1,224 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io';
import 'package:flutter/services.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:in_app_purchase_storekit/in_app_purchase_storekit.dart';
import 'package:in_app_purchase_storekit/src/channel.dart';
import 'package:in_app_purchase_storekit/store_kit_wrappers.dart';
import '../store_kit_wrappers/sk_test_stub_objects.dart';
class FakeStoreKitPlatform {
FakeStoreKitPlatform() {
_ambiguate(TestDefaultBinaryMessengerBinding.instance)!
.defaultBinaryMessenger
.setMockMethodCallHandler(channel, onMethodCall);
}
// pre-configured store information
String? receiptData;
late Set<String> validProductIDs;
late Map<String, SKProductWrapper> validProducts;
late List<SKPaymentTransactionWrapper> transactions;
late List<SKPaymentTransactionWrapper> finishedTransactions;
late bool testRestoredTransactionsNull;
late bool testTransactionFail;
late int testTransactionCancel;
PlatformException? queryProductException;
PlatformException? restoreException;
SKError? testRestoredError;
bool queueIsActive = false;
Map<String, dynamic> discountReceived = <String, dynamic>{};
void reset() {
transactions = <SKPaymentTransactionWrapper>[];
receiptData = 'dummy base64data';
validProductIDs = <String>{'123', '456'};
validProducts = <String, SKProductWrapper>{};
for (final String validID in validProductIDs) {
final Map<String, dynamic> productWrapperMap =
buildProductMap(dummyProductWrapper);
productWrapperMap['productIdentifier'] = validID;
if (validID == '456') {
productWrapperMap['priceLocale'] = buildLocaleMap(noSymbolLocale);
}
validProducts[validID] = SKProductWrapper.fromJson(productWrapperMap);
}
finishedTransactions = <SKPaymentTransactionWrapper>[];
testRestoredTransactionsNull = false;
testTransactionFail = false;
testTransactionCancel = -1;
queryProductException = null;
restoreException = null;
testRestoredError = null;
queueIsActive = false;
discountReceived = <String, dynamic>{};
}
SKPaymentTransactionWrapper createPendingTransaction(String id,
{int quantity = 1}) {
return SKPaymentTransactionWrapper(
transactionIdentifier: '',
payment: SKPaymentWrapper(productIdentifier: id, quantity: quantity),
transactionState: SKPaymentTransactionStateWrapper.purchasing,
transactionTimeStamp: 123123.121,
);
}
SKPaymentTransactionWrapper createPurchasedTransaction(
String productId, String transactionId,
{int quantity = 1}) {
return SKPaymentTransactionWrapper(
payment:
SKPaymentWrapper(productIdentifier: productId, quantity: quantity),
transactionState: SKPaymentTransactionStateWrapper.purchased,
transactionTimeStamp: 123123.121,
transactionIdentifier: transactionId);
}
SKPaymentTransactionWrapper createFailedTransaction(String productId,
{int quantity = 1}) {
return SKPaymentTransactionWrapper(
transactionIdentifier: '',
payment:
SKPaymentWrapper(productIdentifier: productId, quantity: quantity),
transactionState: SKPaymentTransactionStateWrapper.failed,
transactionTimeStamp: 123123.121,
error: const SKError(
code: 0,
domain: 'ios_domain',
userInfo: <String, Object>{'message': 'an error message'}));
}
SKPaymentTransactionWrapper createCanceledTransaction(
String productId, int errorCode,
{int quantity = 1}) {
return SKPaymentTransactionWrapper(
transactionIdentifier: '',
payment:
SKPaymentWrapper(productIdentifier: productId, quantity: quantity),
transactionState: SKPaymentTransactionStateWrapper.failed,
transactionTimeStamp: 123123.121,
error: SKError(
code: errorCode,
domain: 'ios_domain',
userInfo: const <String, Object>{'message': 'an error message'}));
}
SKPaymentTransactionWrapper createRestoredTransaction(
String productId, String transactionId,
{int quantity = 1}) {
return SKPaymentTransactionWrapper(
payment:
SKPaymentWrapper(productIdentifier: productId, quantity: quantity),
transactionState: SKPaymentTransactionStateWrapper.restored,
transactionTimeStamp: 123123.121,
transactionIdentifier: transactionId);
}
Future<dynamic> onMethodCall(MethodCall call) {
switch (call.method) {
case '-[SKPaymentQueue canMakePayments:]':
return Future<bool>.value(true);
case '-[InAppPurchasePlugin startProductRequest:result:]':
if (queryProductException != null) {
throw queryProductException!;
}
final List<String> productIDS =
List.castFrom<dynamic, String>(call.arguments as List<dynamic>);
final List<String> invalidFound = <String>[];
final List<SKProductWrapper> products = <SKProductWrapper>[];
for (final String productID in productIDS) {
if (!validProductIDs.contains(productID)) {
invalidFound.add(productID);
} else {
products.add(validProducts[productID]!);
}
}
final SkProductResponseWrapper response = SkProductResponseWrapper(
products: products, invalidProductIdentifiers: invalidFound);
return Future<Map<String, dynamic>>.value(
buildProductResponseMap(response));
case '-[InAppPurchasePlugin restoreTransactions:result:]':
if (restoreException != null) {
throw restoreException!;
}
if (testRestoredError != null) {
InAppPurchaseStoreKitPlatform.observer
.restoreCompletedTransactionsFailed(error: testRestoredError!);
return Future<void>.sync(() {});
}
if (!testRestoredTransactionsNull) {
InAppPurchaseStoreKitPlatform.observer
.updatedTransactions(transactions: transactions);
}
InAppPurchaseStoreKitPlatform.observer
.paymentQueueRestoreCompletedTransactionsFinished();
return Future<void>.sync(() {});
case '-[InAppPurchasePlugin retrieveReceiptData:result:]':
if (receiptData != null) {
return Future<String>.value(receiptData);
} else {
throw PlatformException(code: 'no_receipt_data');
}
case '-[InAppPurchasePlugin refreshReceipt:result:]':
receiptData = 'refreshed receipt data';
return Future<void>.sync(() {});
case '-[InAppPurchasePlugin addPayment:result:]':
final Map<String, Object?> arguments = _getArgumentDictionary(call);
final String id = arguments['productIdentifier']! as String;
final int quantity = arguments['quantity']! as int;
// Keep the received paymentDiscount parameter when testing payment with discount.
if (arguments['applicationUsername']! == 'userWithDiscount') {
final Map<dynamic, dynamic>? discountArgument =
arguments['paymentDiscount'] as Map<dynamic, dynamic>?;
if (discountArgument != null) {
discountReceived = discountArgument.cast<String, dynamic>();
} else {
discountReceived = <String, dynamic>{};
}
}
final SKPaymentTransactionWrapper transaction =
createPendingTransaction(id, quantity: quantity);
transactions.add(transaction);
InAppPurchaseStoreKitPlatform.observer.updatedTransactions(
transactions: <SKPaymentTransactionWrapper>[transaction]);
sleep(const Duration(milliseconds: 30));
if (testTransactionFail) {
final SKPaymentTransactionWrapper transactionFailed =
createFailedTransaction(id, quantity: quantity);
InAppPurchaseStoreKitPlatform.observer.updatedTransactions(
transactions: <SKPaymentTransactionWrapper>[transactionFailed]);
} else if (testTransactionCancel > 0) {
final SKPaymentTransactionWrapper transactionCanceled =
createCanceledTransaction(id, testTransactionCancel,
quantity: quantity);
InAppPurchaseStoreKitPlatform.observer.updatedTransactions(
transactions: <SKPaymentTransactionWrapper>[transactionCanceled]);
} else {
final SKPaymentTransactionWrapper transactionFinished =
createPurchasedTransaction(
id, transaction.transactionIdentifier ?? '',
quantity: quantity);
InAppPurchaseStoreKitPlatform.observer.updatedTransactions(
transactions: <SKPaymentTransactionWrapper>[transactionFinished]);
}
break;
case '-[InAppPurchasePlugin finishTransaction:result:]':
final Map<String, Object?> arguments = _getArgumentDictionary(call);
finishedTransactions.add(createPurchasedTransaction(
arguments['productIdentifier']! as String,
arguments['transactionIdentifier']! as String,
quantity: transactions.first.payment.quantity));
break;
case '-[SKPaymentQueue startObservingTransactionQueue]':
queueIsActive = true;
break;
case '-[SKPaymentQueue stopObservingTransactionQueue]':
queueIsActive = false;
break;
}
return Future<void>.sync(() {});
}
/// Returns the arguments of [call] as typed string-keyed Map.
///
/// This does not do any type validation, so is only safe to call if the
/// arguments are known to be a map.
Map<String, Object?> _getArgumentDictionary(MethodCall call) {
return (call.arguments as Map<Object?, Object?>).cast<String, Object?>();
}
}
/// This allows a value of type T or T? to be treated as a value of type T?.
///
/// We use this so that APIs that have become non-nullable can still be used
/// with `!` and `?` on the stable branch.
T? _ambiguate<T>(T? value) => value;
| plugins/packages/in_app_purchase/in_app_purchase_storekit/test/fakes/fake_storekit_platform.dart/0 | {
"file_path": "plugins/packages/in_app_purchase/in_app_purchase_storekit/test/fakes/fake_storekit_platform.dart",
"repo_id": "plugins",
"token_count": 3779
} | 1,225 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#import <Flutter/Flutter.h>
/// A plugin for Flutter that allows Flutter to load images in a platform
/// specific way on iOS.
@interface IosPlatformImagesPlugin : NSObject <FlutterPlugin>
@end
| plugins/packages/ios_platform_images/ios/Classes/IosPlatformImagesPlugin.h/0 | {
"file_path": "plugins/packages/ios_platform_images/ios/Classes/IosPlatformImagesPlugin.h",
"repo_id": "plugins",
"token_count": 98
} | 1,226 |
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item>
<shape android:shape="oval">
<solid android:color="@color/warning_color"/>
<size
android:width="40dp"
android:height="40dp"/>
</shape>
</item>
<item android:drawable="@drawable/ic_priority_high_white_24dp"
android:bottom="8dp"
android:left="8dp"
android:right="8dp"
android:top="8dp"/>
</layer-list>
| plugins/packages/local_auth/local_auth_android/android/src/main/res/drawable/fingerprint_warning_icon.xml/0 | {
"file_path": "plugins/packages/local_auth/local_auth_android/android/src/main/res/drawable/fingerprint_warning_icon.xml",
"repo_id": "plugins",
"token_count": 215
} | 1,227 |
name: local_auth_android
description: Android implementation of the local_auth plugin.
repository: https://github.com/flutter/plugins/tree/main/packages/local_auth/local_auth_android
issue_tracker: https://github.com/flutter/flutter/issues?q=is%3Aissue+is%3Aopen+label%3A%22p%3A+local_auth%22
version: 1.0.18
environment:
sdk: ">=2.14.0 <3.0.0"
flutter: ">=3.0.0"
flutter:
plugin:
implements: local_auth
platforms:
android:
package: io.flutter.plugins.localauth
pluginClass: LocalAuthPlugin
dartPluginClass: LocalAuthAndroid
dependencies:
flutter:
sdk: flutter
flutter_plugin_android_lifecycle: ^2.0.1
intl: ">=0.17.0 <0.19.0"
local_auth_platform_interface: ^1.0.1
dev_dependencies:
flutter_test:
sdk: flutter
| plugins/packages/local_auth/local_auth_android/pubspec.yaml/0 | {
"file_path": "plugins/packages/local_auth/local_auth_android/pubspec.yaml",
"repo_id": "plugins",
"token_count": 323
} | 1,228 |
## NEXT
* Updates minimum Flutter version to 3.0.
## 1.0.5
* Switches internal implementation to Pigeon.
## 1.0.4
* Updates imports for `prefer_relative_imports`.
* Updates minimum Flutter version to 2.10.
## 1.0.3
* Fixes avoid_redundant_argument_values lint warnings and minor typos.
## 1.0.2
* Updates `local_auth_platform_interface` constraint to the correct minimum
version.
## 1.0.1
* Updates references to the obsolete master branch.
## 1.0.0
* Initial release of Windows support.
| plugins/packages/local_auth/local_auth_windows/CHANGELOG.md/0 | {
"file_path": "plugins/packages/local_auth/local_auth_windows/CHANGELOG.md",
"repo_id": "plugins",
"token_count": 166
} | 1,229 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io';
import 'package:flutter_test/flutter_test.dart';
import 'package:integration_test/integration_test.dart';
import 'package:path_provider_platform_interface/path_provider_platform_interface.dart';
void main() {
IntegrationTestWidgetsFlutterBinding.ensureInitialized();
testWidgets('getTemporaryDirectory', (WidgetTester tester) async {
final PathProviderPlatform provider = PathProviderPlatform.instance;
final String? result = await provider.getTemporaryPath();
_verifySampleFile(result, 'temporaryDirectory');
});
testWidgets('getApplicationDocumentsDirectory', (WidgetTester tester) async {
final PathProviderPlatform provider = PathProviderPlatform.instance;
final String? result = await provider.getApplicationDocumentsPath();
_verifySampleFile(result, 'applicationDocuments');
});
testWidgets('getApplicationSupportDirectory', (WidgetTester tester) async {
final PathProviderPlatform provider = PathProviderPlatform.instance;
final String? result = await provider.getApplicationSupportPath();
_verifySampleFile(result, 'applicationSupport');
});
testWidgets('getLibraryDirectory', (WidgetTester tester) async {
final PathProviderPlatform provider = PathProviderPlatform.instance;
expect(() => provider.getLibraryPath(),
throwsA(isInstanceOf<UnsupportedError>()));
});
testWidgets('getExternalStorageDirectory', (WidgetTester tester) async {
final PathProviderPlatform provider = PathProviderPlatform.instance;
final String? result = await provider.getExternalStoragePath();
_verifySampleFile(result, 'externalStorage');
});
testWidgets('getExternalCacheDirectories', (WidgetTester tester) async {
final PathProviderPlatform provider = PathProviderPlatform.instance;
final List<String>? directories = await provider.getExternalCachePaths();
expect(directories, isNotNull);
for (final String result in directories!) {
_verifySampleFile(result, 'externalCache');
}
});
final List<StorageDirectory?> allDirs = <StorageDirectory?>[
null,
StorageDirectory.music,
StorageDirectory.podcasts,
StorageDirectory.ringtones,
StorageDirectory.alarms,
StorageDirectory.notifications,
StorageDirectory.pictures,
StorageDirectory.movies,
];
for (final StorageDirectory? type in allDirs) {
testWidgets('getExternalStorageDirectories (type: $type)',
(WidgetTester tester) async {
final PathProviderPlatform provider = PathProviderPlatform.instance;
final List<String>? directories =
await provider.getExternalStoragePaths(type: type);
expect(directories, isNotNull);
expect(directories, isNotEmpty);
for (final String result in directories!) {
_verifySampleFile(result, '$type');
}
});
}
}
/// Verify a file called [name] in [directoryPath] by recreating it with test
/// contents when necessary.
void _verifySampleFile(String? directoryPath, String name) {
expect(directoryPath, isNotNull);
if (directoryPath == null) {
return;
}
final Directory directory = Directory(directoryPath);
final File file = File('${directory.path}${Platform.pathSeparator}$name');
if (file.existsSync()) {
file.deleteSync();
expect(file.existsSync(), isFalse);
}
file.writeAsStringSync('Hello world!');
expect(file.readAsStringSync(), 'Hello world!');
expect(directory.listSync(), isNotEmpty);
file.deleteSync();
}
| plugins/packages/path_provider/path_provider_android/example/integration_test/path_provider_test.dart/0 | {
"file_path": "plugins/packages/path_provider/path_provider_android/example/integration_test/path_provider_test.dart",
"repo_id": "plugins",
"token_count": 1096
} | 1,230 |
#include "../../Flutter/Flutter-Debug.xcconfig"
#include "Warnings.xcconfig"
| plugins/packages/path_provider/path_provider_foundation/example/macos/Runner/Configs/Debug.xcconfig/0 | {
"file_path": "plugins/packages/path_provider/path_provider_foundation/example/macos/Runner/Configs/Debug.xcconfig",
"repo_id": "plugins",
"token_count": 32
} | 1,231 |
# quick\_actions\_android
The Android implementation of [`quick_actions`][1].
## Usage
This package is [endorsed][2], which means you can simply use `quick_actions`
normally. This package will be automatically included in your app when you do.
## Contributing
If you would like to contribute to the plugin, check out our [contribution guide][3].
[1]: https://pub.dev/packages/quick_actions
[2]: https://flutter.dev/docs/development/packages-and-plugins/developing-packages#endorsed-federated-plugin
[3]: https://github.com/flutter/plugins/blob/main/CONTRIBUTING.md
| plugins/packages/quick_actions/quick_actions_android/README.md/0 | {
"file_path": "plugins/packages/quick_actions/quick_actions_android/README.md",
"repo_id": "plugins",
"token_count": 173
} | 1,232 |
name: quick_actions_android
description: An implementation for the Android platform of the Flutter `quick_actions` plugin.
repository: https://github.com/flutter/plugins/tree/main/packages/quick_actions/quick_actions_android
issue_tracker: https://github.com/flutter/flutter/issues?q=is%3Aissue+is%3Aopen+label%3A%22p%3A+in_app_purchase%22
version: 1.0.0
environment:
sdk: ">=2.15.0 <3.0.0"
flutter: ">=3.0.0"
flutter:
plugin:
implements: quick_actions
platforms:
android:
package: io.flutter.plugins.quickactions
pluginClass: QuickActionsPlugin
dartPluginClass: QuickActionsAndroid
dependencies:
flutter:
sdk: flutter
quick_actions_platform_interface: ^1.0.0
dev_dependencies:
flutter_test:
sdk: flutter
integration_test:
sdk: flutter
plugin_platform_interface: ^2.1.2
| plugins/packages/quick_actions/quick_actions_android/pubspec.yaml/0 | {
"file_path": "plugins/packages/quick_actions/quick_actions_android/pubspec.yaml",
"repo_id": "plugins",
"token_count": 327
} | 1,233 |
group 'io.flutter.plugins.sharedpreferences'
version '1.0-SNAPSHOT'
buildscript {
repositories {
google()
mavenCentral()
}
dependencies {
classpath 'com.android.tools.build:gradle:7.2.2'
}
}
rootProject.allprojects {
repositories {
google()
mavenCentral()
}
}
allprojects {
gradle.projectsEvaluated {
tasks.withType(JavaCompile) {
options.compilerArgs << "-Xlint:unchecked" << "-Xlint:deprecation"
}
}
}
apply plugin: 'com.android.library'
android {
compileSdkVersion 31
defaultConfig {
minSdkVersion 16
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
lintOptions {
disable 'AndroidGradlePluginVersion', 'InvalidPackage', 'GradleDependency'
baseline file("lint-baseline.xml")
}
dependencies {
testImplementation 'junit:junit:4.13.2'
testImplementation 'org.mockito:mockito-inline:5.0.0'
}
testOptions {
unitTests.includeAndroidResources = true
unitTests.returnDefaultValues = true
unitTests.all {
testLogging {
events "passed", "skipped", "failed", "standardOut", "standardError"
outputs.upToDateWhen {false}
showStandardStreams = true
}
}
}
}
| plugins/packages/shared_preferences/shared_preferences_android/android/build.gradle/0 | {
"file_path": "plugins/packages/shared_preferences/shared_preferences_android/android/build.gradle",
"repo_id": "plugins",
"token_count": 617
} | 1,234 |
name: shared_preferences_foundation
description: iOS and macOS implementation of the shared_preferences plugin.
repository: https://github.com/flutter/plugins/tree/main/packages/shared_preferences/shared_preferences_foundation
issue_tracker: https://github.com/flutter/flutter/issues?q=is%3Aissue+is%3Aopen+label%3A%22p%3A+shared_preferences%22
version: 2.1.3
environment:
sdk: ">=2.12.0 <3.0.0"
flutter: ">=3.0.0"
flutter:
plugin:
implements: shared_preferences
platforms:
ios:
pluginClass: SharedPreferencesPlugin
dartPluginClass: SharedPreferencesFoundation
sharedDarwinSource: true
macos:
pluginClass: SharedPreferencesPlugin
dartPluginClass: SharedPreferencesFoundation
sharedDarwinSource: true
dependencies:
flutter:
sdk: flutter
shared_preferences_platform_interface: ^2.0.0
dev_dependencies:
flutter_test:
sdk: flutter
pigeon: ^5.0.0
| plugins/packages/shared_preferences/shared_preferences_foundation/pubspec.yaml/0 | {
"file_path": "plugins/packages/shared_preferences/shared_preferences_foundation/pubspec.yaml",
"repo_id": "plugins",
"token_count": 365
} | 1,235 |
name: shared_preferences_platform_interface
description: A common platform interface for the shared_preferences plugin.
repository: https://github.com/flutter/plugins/tree/main/packages/shared_preferences/shared_preferences_platform_interface
issue_tracker: https://github.com/flutter/flutter/issues?q=is%3Aissue+is%3Aopen+label%3A%22p%3A+shared_preferences%22
version: 2.1.0
environment:
sdk: ">=2.12.0 <3.0.0"
flutter: ">=3.0.0"
dependencies:
flutter:
sdk: flutter
plugin_platform_interface: ^2.1.0
dev_dependencies:
flutter_test:
sdk: flutter
| plugins/packages/shared_preferences/shared_preferences_platform_interface/pubspec.yaml/0 | {
"file_path": "plugins/packages/shared_preferences/shared_preferences_platform_interface/pubspec.yaml",
"repo_id": "plugins",
"token_count": 219
} | 1,236 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_test/flutter_test.dart';
import 'package:plugin_platform_interface/plugin_platform_interface.dart';
import 'package:url_launcher_platform_interface/link.dart';
import 'package:url_launcher_platform_interface/url_launcher_platform_interface.dart';
class MockUrlLauncher extends Fake
with MockPlatformInterfaceMixin
implements UrlLauncherPlatform {
String? url;
PreferredLaunchMode? launchMode;
bool? useSafariVC;
bool? useWebView;
bool? enableJavaScript;
bool? enableDomStorage;
bool? universalLinksOnly;
Map<String, String>? headers;
String? webOnlyWindowName;
bool? response;
bool closeWebViewCalled = false;
bool canLaunchCalled = false;
bool launchCalled = false;
// ignore: use_setters_to_change_properties
void setCanLaunchExpectations(String url) {
this.url = url;
}
void setLaunchExpectations({
required String url,
PreferredLaunchMode? launchMode,
bool? useSafariVC,
bool? useWebView,
required bool enableJavaScript,
required bool enableDomStorage,
required bool universalLinksOnly,
required Map<String, String> headers,
required String? webOnlyWindowName,
}) {
this.url = url;
this.launchMode = launchMode;
this.useSafariVC = useSafariVC;
this.useWebView = useWebView;
this.enableJavaScript = enableJavaScript;
this.enableDomStorage = enableDomStorage;
this.universalLinksOnly = universalLinksOnly;
this.headers = headers;
this.webOnlyWindowName = webOnlyWindowName;
}
// ignore: use_setters_to_change_properties
void setResponse(bool response) {
this.response = response;
}
@override
LinkDelegate? get linkDelegate => null;
@override
Future<bool> canLaunch(String url) async {
expect(url, this.url);
canLaunchCalled = true;
return response!;
}
@override
Future<bool> launch(
String url, {
required bool useSafariVC,
required bool useWebView,
required bool enableJavaScript,
required bool enableDomStorage,
required bool universalLinksOnly,
required Map<String, String> headers,
String? webOnlyWindowName,
}) async {
expect(url, this.url);
expect(useSafariVC, this.useSafariVC);
expect(useWebView, this.useWebView);
expect(enableJavaScript, this.enableJavaScript);
expect(enableDomStorage, this.enableDomStorage);
expect(universalLinksOnly, this.universalLinksOnly);
expect(headers, this.headers);
expect(webOnlyWindowName, this.webOnlyWindowName);
launchCalled = true;
return response!;
}
@override
Future<bool> launchUrl(String url, LaunchOptions options) async {
expect(url, this.url);
expect(options.mode, launchMode);
expect(options.webViewConfiguration.enableJavaScript, enableJavaScript);
expect(options.webViewConfiguration.enableDomStorage, enableDomStorage);
expect(options.webViewConfiguration.headers, headers);
expect(options.webOnlyWindowName, webOnlyWindowName);
launchCalled = true;
return response!;
}
@override
Future<void> closeWebView() async {
closeWebViewCalled = true;
}
}
| plugins/packages/url_launcher/url_launcher/test/mocks/mock_url_launcher_platform.dart/0 | {
"file_path": "plugins/packages/url_launcher/url_launcher/test/mocks/mock_url_launcher_platform.dart",
"repo_id": "plugins",
"token_count": 1079
} | 1,237 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.urllauncher;
import static org.junit.Assert.assertEquals;
import java.util.Collections;
import org.junit.Test;
public class WebViewActivityTest {
@Test
public void extractHeaders_returnsEmptyMapWhenHeadersBundleNull() {
assertEquals(WebViewActivity.extractHeaders(null), Collections.emptyMap());
}
}
| plugins/packages/url_launcher/url_launcher_android/android/src/test/java/io/flutter/plugins/urllauncher/WebViewActivityTest.java/0 | {
"file_path": "plugins/packages/url_launcher/url_launcher_android/android/src/test/java/io/flutter/plugins/urllauncher/WebViewActivityTest.java",
"repo_id": "plugins",
"token_count": 153
} | 1,238 |
name: url_launcher_example
description: Demonstrates how to use the url_launcher plugin.
publish_to: none
environment:
sdk: '>=2.18.0 <3.0.0'
flutter: ">=3.3.0"
dependencies:
flutter:
sdk: flutter
url_launcher_ios:
# When depending on this package from a real application you should use:
# url_launcher_ios: ^x.y.z
# See https://dart.dev/tools/pub/dependencies#version-constraints
# The example app is bundled with the plugin so we use a path dependency on
# the parent directory to use the current plugin's version.
path: ../
url_launcher_platform_interface: ^2.0.3
dev_dependencies:
flutter_driver:
sdk: flutter
flutter_test:
sdk: flutter
integration_test:
sdk: flutter
mockito: ^5.0.0
plugin_platform_interface: ^2.0.0
flutter:
uses-material-design: true
| plugins/packages/url_launcher/url_launcher_ios/example/pubspec.yaml/0 | {
"file_path": "plugins/packages/url_launcher/url_launcher_ios/example/pubspec.yaml",
"repo_id": "plugins",
"token_count": 315
} | 1,239 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include <flutter_linux/flutter_linux.h>
#include <gmock/gmock.h>
#include <gtest/gtest.h>
#include <memory>
#include <string>
#include "include/url_launcher_linux/url_launcher_plugin.h"
#include "url_launcher_plugin_private.h"
namespace url_launcher_plugin {
namespace test {
TEST(UrlLauncherPlugin, CanLaunchSuccess) {
g_autoptr(FlValue) args = fl_value_new_map();
fl_value_set_string_take(args, "url",
fl_value_new_string("https://flutter.dev"));
g_autoptr(FlMethodResponse) response = can_launch(nullptr, args);
ASSERT_NE(response, nullptr);
ASSERT_TRUE(FL_IS_METHOD_SUCCESS_RESPONSE(response));
g_autoptr(FlValue) expected = fl_value_new_bool(true);
EXPECT_TRUE(fl_value_equal(fl_method_success_response_get_result(
FL_METHOD_SUCCESS_RESPONSE(response)),
expected));
}
TEST(UrlLauncherPlugin, CanLaunchFailureUnhandled) {
g_autoptr(FlValue) args = fl_value_new_map();
fl_value_set_string_take(args, "url", fl_value_new_string("madeup:scheme"));
g_autoptr(FlMethodResponse) response = can_launch(nullptr, args);
ASSERT_NE(response, nullptr);
ASSERT_TRUE(FL_IS_METHOD_SUCCESS_RESPONSE(response));
g_autoptr(FlValue) expected = fl_value_new_bool(false);
EXPECT_TRUE(fl_value_equal(fl_method_success_response_get_result(
FL_METHOD_SUCCESS_RESPONSE(response)),
expected));
}
TEST(UrlLauncherPlugin, CanLaunchFileSuccess) {
g_autoptr(FlValue) args = fl_value_new_map();
fl_value_set_string_take(args, "url", fl_value_new_string("file:///"));
g_autoptr(FlMethodResponse) response = can_launch(nullptr, args);
ASSERT_NE(response, nullptr);
ASSERT_TRUE(FL_IS_METHOD_SUCCESS_RESPONSE(response));
g_autoptr(FlValue) expected = fl_value_new_bool(true);
EXPECT_TRUE(fl_value_equal(fl_method_success_response_get_result(
FL_METHOD_SUCCESS_RESPONSE(response)),
expected));
}
TEST(UrlLauncherPlugin, CanLaunchFailureInvalidFileExtension) {
g_autoptr(FlValue) args = fl_value_new_map();
fl_value_set_string_take(
args, "url", fl_value_new_string("file:///madeup.madeupextension"));
g_autoptr(FlMethodResponse) response = can_launch(nullptr, args);
ASSERT_NE(response, nullptr);
ASSERT_TRUE(FL_IS_METHOD_SUCCESS_RESPONSE(response));
g_autoptr(FlValue) expected = fl_value_new_bool(false);
EXPECT_TRUE(fl_value_equal(fl_method_success_response_get_result(
FL_METHOD_SUCCESS_RESPONSE(response)),
expected));
}
// For consistency with the established mobile implementations,
// an invalid URL should return false, not an error.
TEST(UrlLauncherPlugin, CanLaunchFailureInvalidUrl) {
g_autoptr(FlValue) args = fl_value_new_map();
fl_value_set_string_take(args, "url", fl_value_new_string(""));
g_autoptr(FlMethodResponse) response = can_launch(nullptr, args);
ASSERT_NE(response, nullptr);
ASSERT_TRUE(FL_IS_METHOD_SUCCESS_RESPONSE(response));
g_autoptr(FlValue) expected = fl_value_new_bool(false);
EXPECT_TRUE(fl_value_equal(fl_method_success_response_get_result(
FL_METHOD_SUCCESS_RESPONSE(response)),
expected));
}
} // namespace test
} // namespace url_launcher_plugin
| plugins/packages/url_launcher/url_launcher_linux/linux/test/url_launcher_linux_test.cc/0 | {
"file_path": "plugins/packages/url_launcher/url_launcher_linux/linux/test/url_launcher_linux_test.cc",
"repo_id": "plugins",
"token_count": 1520
} | 1,240 |
targets:
$default:
sources:
- integration_test/*.dart
- lib/$lib$
- $package$
| plugins/packages/url_launcher/url_launcher_web/example/build.yaml/0 | {
"file_path": "plugins/packages/url_launcher/url_launcher_web/example/build.yaml",
"repo_id": "plugins",
"token_count": 51
} | 1,241 |
// Copyright 2017 Workiva Inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
// //////////////////////////////////////////////////////////
//
// This file is a stripped down, and slightly modified version of
// package:platform_detect's.
//
// Original version here: https://github.com/Workiva/platform_detect
//
// //////////////////////////////////////////////////////////
import 'dart:html' as html show Navigator;
/// Determines if the `navigator` is Safari.
bool navigatorIsSafari(html.Navigator navigator) {
// An web view running in an iOS app does not have a 'Version/X.X.X' string in the appVersion
final String vendor = navigator.vendor;
final String appVersion = navigator.appVersion;
return vendor != null &&
vendor.contains('Apple') &&
appVersion != null &&
appVersion.contains('Version');
}
| plugins/packages/url_launcher/url_launcher_web/lib/src/third_party/platform_detect/browser.dart/0 | {
"file_path": "plugins/packages/url_launcher/url_launcher_web/lib/src/third_party/platform_detect/browser.dart",
"repo_id": "plugins",
"token_count": 361
} | 1,242 |
group 'io.flutter.plugins.videoplayer'
version '1.0-SNAPSHOT'
def args = ["-Xlint:deprecation","-Xlint:unchecked","-Werror"]
buildscript {
repositories {
google()
mavenCentral()
}
dependencies {
classpath 'com.android.tools.build:gradle:7.2.1'
}
}
rootProject.allprojects {
repositories {
google()
mavenCentral()
}
}
project.getTasks().withType(JavaCompile){
options.compilerArgs.addAll(args)
}
apply plugin: 'com.android.library'
android {
compileSdkVersion 31
defaultConfig {
minSdkVersion 16
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
lintOptions {
disable 'AndroidGradlePluginVersion', 'InvalidPackage', 'GradleDependency'
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
dependencies {
implementation 'com.google.android.exoplayer:exoplayer-core:2.18.1'
implementation 'com.google.android.exoplayer:exoplayer-hls:2.18.1'
implementation 'com.google.android.exoplayer:exoplayer-dash:2.18.1'
implementation 'com.google.android.exoplayer:exoplayer-smoothstreaming:2.18.1'
testImplementation 'junit:junit:4.13.2'
testImplementation 'androidx.test:core:1.3.0'
testImplementation 'org.mockito:mockito-inline:5.0.0'
testImplementation 'org.robolectric:robolectric:4.8.1'
}
testOptions {
unitTests.includeAndroidResources = true
unitTests.returnDefaultValues = true
unitTests.all {
testLogging {
events "passed", "skipped", "failed", "standardOut", "standardError"
outputs.upToDateWhen {false}
showStandardStreams = true
}
}
}
}
| plugins/packages/video_player/video_player_android/android/build.gradle/0 | {
"file_path": "plugins/packages/video_player/video_player_android/android/build.gradle",
"repo_id": "plugins",
"token_count": 805
} | 1,243 |
## NEXT
* Updates minimum Flutter version to 3.0.
## 2.3.8
* Adds compatibilty with version 6.0 of the platform interface.
* Fixes file URI construction in example.
* Updates code for new analysis options.
* Adds an integration test for a bug where the aspect ratios of some HLS videos are incorrectly inverted.
* Removes an unnecessary override in example code.
## 2.3.7
* Fixes a bug where the aspect ratio of some HLS videos are incorrectly inverted.
* Updates code for `no_leading_underscores_for_local_identifiers` lint.
## 2.3.6
* Fixes a bug in iOS 16 where videos from protected live streams are not shown.
* Updates minimum Flutter version to 2.10.
* Fixes violations of new analysis option use_named_constants.
* Fixes avoid_redundant_argument_values lint warnings and minor typos.
* Ignores unnecessary import warnings in preparation for [upcoming Flutter changes](https://github.com/flutter/flutter/pull/106316).
## 2.3.5
* Updates references to the obsolete master branch.
## 2.3.4
* Removes unnecessary imports.
* Fixes library_private_types_in_public_api, sort_child_properties_last and use_key_in_widget_constructors
lint warnings.
## 2.3.3
* Fix XCUITest based on the new voice over announcement for tooltips.
See: https://github.com/flutter/flutter/pull/87684
## 2.3.2
* Applies the standardized transform for videos with different orientations.
## 2.3.1
* Renames internal method channels to avoid potential confusion with the
default implementation's method channel.
* Updates Pigeon to 2.0.1.
## 2.3.0
* Updates Pigeon to ^1.0.16.
## 2.2.18
* Wait to initialize m3u8 videos until size is set, fixing aspect ratio.
* Adjusts test timeouts for network-dependent native tests to avoid flake.
## 2.2.17
* Splits from `video_player` as a federated implementation.
| plugins/packages/video_player/video_player_avfoundation/CHANGELOG.md/0 | {
"file_path": "plugins/packages/video_player/video_player_avfoundation/CHANGELOG.md",
"repo_id": "plugins",
"token_count": 533
} | 1,244 |
# video_player_web
The web implementation of [`video_player`][1].
## Usage
This package is [endorsed](https://flutter.dev/docs/development/packages-and-plugins/developing-packages#endorsed-federated-plugin),
which means you can simply use `video_player` normally. This package will be
automatically included in your app when you do.
## Limitations on the Web platform
Video playback on the Web platform has some limitations that might surprise developers
more familiar with mobile/desktop targets.
In no particular order:
### dart:io
The web platform does **not** suppport `dart:io`, so attempts to create a `VideoPlayerController.file`
will throw an `UnimplementedError`.
### Autoplay
Attempts to start playing videos with an audio track (or not muted) without user
interaction with the site ("user activation") will be prohibited by the browser
and cause runtime errors in JS.
See also:
* [Autoplay policy in Chrome](https://developer.chrome.com/blog/autoplay/)
* MDN > [Autoplay guide for media and Web Audio APIs](https://developer.mozilla.org/en-US/docs/Web/Media/Autoplay_guide)
* Delivering Video Content for Safari > [Enable Video Autoplay](https://developer.apple.com/documentation/webkit/delivering_video_content_for_safari#3030251)
* More info about "user activation", in general:
* [Making user activation consistent across APIs](https://developer.chrome.com/blog/user-activation)
* HTML Spec: [Tracking user activation](https://html.spec.whatwg.org/multipage/interaction.html#sticky-activation)
### Some videos restart when using the seek bar/progress bar/scrubber
Certain videos will rewind to the beginning when users attempt to `seekTo` (change
the progress/scrub to) another position, instead of jumping to the desired position.
Once the video is fully stored in the browser cache, seeking will work fine after
a full page reload.
The most common explanation for this issue is that the server where the video is
stored doesn't support [HTTP range requests](https://developer.mozilla.org/en-US/docs/Web/HTTP/Range_requests).
> **NOTE:** Flutter web's local server (the one that powers `flutter run`) **DOES NOT** support
> range requests, so all video **assets** in `debug` mode will exhibit this behavior.
See [Issue #49360](https://github.com/flutter/flutter/issues/49360) for more information
on how to diagnose if a server supports range requests or not.
### Mixing audio with other audio sources
The `VideoPlayerOptions.mixWithOthers` option can't be implemented in web, at least
at the moment. If you use this option it will be silently ignored.
## Supported Formats
**Different web browsers support different sets of video codecs.**
### Video codecs?
Check MDN's [**Web video codec guide**](https://developer.mozilla.org/en-US/docs/Web/Media/Formats/Video_codecs)
to learn more about the pros and cons of each video codec.
### What codecs are supported?
Visit [**caniuse.com: 'video format'**](https://caniuse.com/#search=video%20format)
for a breakdown of which browsers support what codecs. You can customize charts
there for the users of your particular website(s).
Here's an abridged version of the data from caniuse, for a Global audience:
#### MPEG-4/H.264
[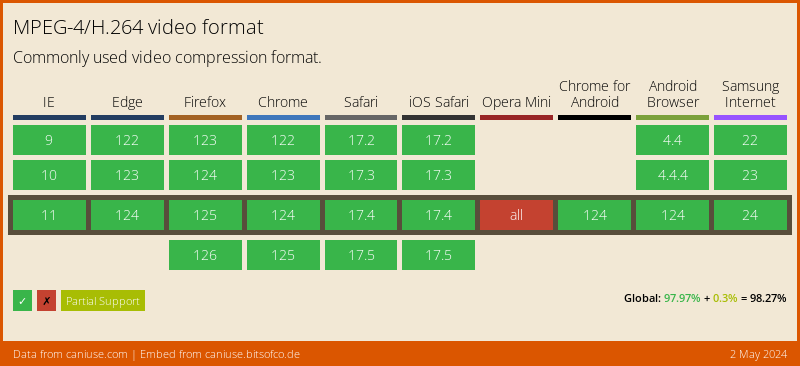](https://caniuse.com/#feat=mpeg4)
#### WebM
[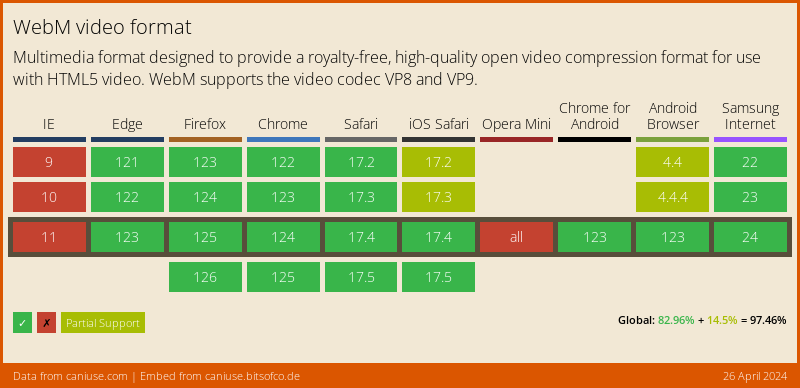](https://caniuse.com/#feat=webm)
#### Ogg/Theora
[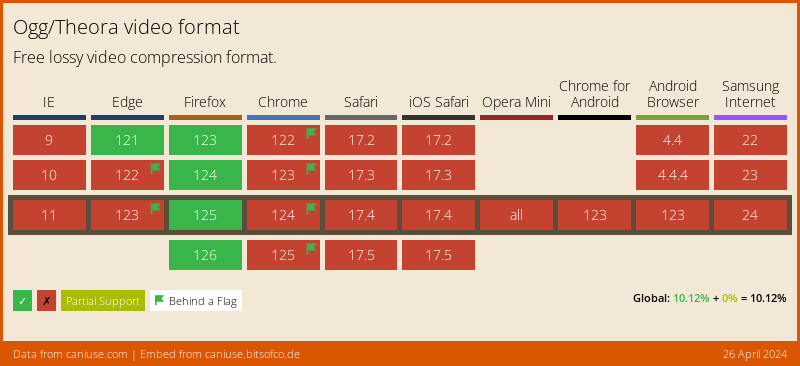](https://caniuse.com/#feat=ogv)
#### AV1
[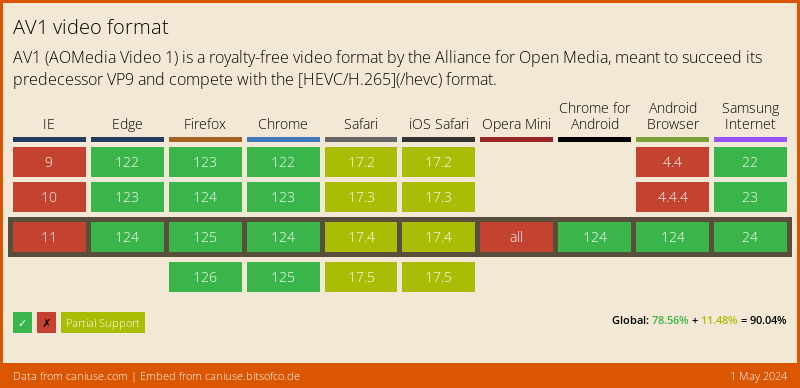](https://caniuse.com/#feat=av1)
#### HEVC/H.265
[](https://caniuse.com/#feat=hevc)
[1]: ../video_player
| plugins/packages/video_player/video_player_web/README.md/0 | {
"file_path": "plugins/packages/video_player/video_player_web/README.md",
"repo_id": "plugins",
"token_count": 1188
} | 1,245 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:html';
import 'package:flutter/material.dart';
import 'package:flutter_web_plugins/flutter_web_plugins.dart';
import 'package:video_player_platform_interface/video_player_platform_interface.dart';
import 'src/shims/dart_ui.dart' as ui;
import 'src/video_player.dart';
/// The web implementation of [VideoPlayerPlatform].
///
/// This class implements the `package:video_player` functionality for the web.
class VideoPlayerPlugin extends VideoPlayerPlatform {
/// Registers this class as the default instance of [VideoPlayerPlatform].
static void registerWith(Registrar registrar) {
VideoPlayerPlatform.instance = VideoPlayerPlugin();
}
// Map of textureId -> VideoPlayer instances
final Map<int, VideoPlayer> _videoPlayers = <int, VideoPlayer>{};
// Simulate the native "textureId".
int _textureCounter = 1;
@override
Future<void> init() async {
return _disposeAllPlayers();
}
@override
Future<void> dispose(int textureId) async {
_player(textureId).dispose();
_videoPlayers.remove(textureId);
return;
}
void _disposeAllPlayers() {
for (final VideoPlayer videoPlayer in _videoPlayers.values) {
videoPlayer.dispose();
}
_videoPlayers.clear();
}
@override
Future<int> create(DataSource dataSource) async {
final int textureId = _textureCounter++;
late String uri;
switch (dataSource.sourceType) {
case DataSourceType.network:
// Do NOT modify the incoming uri, it can be a Blob, and Safari doesn't
// like blobs that have changed.
uri = dataSource.uri ?? '';
break;
case DataSourceType.asset:
String assetUrl = dataSource.asset!;
if (dataSource.package != null && dataSource.package!.isNotEmpty) {
assetUrl = 'packages/${dataSource.package}/$assetUrl';
}
assetUrl = ui.webOnlyAssetManager.getAssetUrl(assetUrl);
uri = assetUrl;
break;
case DataSourceType.file:
return Future<int>.error(UnimplementedError(
'web implementation of video_player cannot play local files'));
case DataSourceType.contentUri:
return Future<int>.error(UnimplementedError(
'web implementation of video_player cannot play content uri'));
}
final VideoElement videoElement = VideoElement()
..id = 'videoElement-$textureId'
..src = uri
..style.border = 'none'
..style.height = '100%'
..style.width = '100%';
// TODO(hterkelsen): Use initialization parameters once they are available
ui.platformViewRegistry.registerViewFactory(
'videoPlayer-$textureId', (int viewId) => videoElement);
final VideoPlayer player = VideoPlayer(videoElement: videoElement)
..initialize();
_videoPlayers[textureId] = player;
return textureId;
}
@override
Future<void> setLooping(int textureId, bool looping) async {
return _player(textureId).setLooping(looping);
}
@override
Future<void> play(int textureId) async {
return _player(textureId).play();
}
@override
Future<void> pause(int textureId) async {
return _player(textureId).pause();
}
@override
Future<void> setVolume(int textureId, double volume) async {
return _player(textureId).setVolume(volume);
}
@override
Future<void> setPlaybackSpeed(int textureId, double speed) async {
return _player(textureId).setPlaybackSpeed(speed);
}
@override
Future<void> seekTo(int textureId, Duration position) async {
return _player(textureId).seekTo(position);
}
@override
Future<Duration> getPosition(int textureId) async {
return _player(textureId).getPosition();
}
@override
Stream<VideoEvent> videoEventsFor(int textureId) {
return _player(textureId).events;
}
// Retrieves a [VideoPlayer] by its internal `id`.
// It must have been created earlier from the [create] method.
VideoPlayer _player(int id) {
return _videoPlayers[id]!;
}
@override
Widget buildView(int textureId) {
return HtmlElementView(viewType: 'videoPlayer-$textureId');
}
/// Sets the audio mode to mix with other sources (ignored)
@override
Future<void> setMixWithOthers(bool mixWithOthers) => Future<void>.value();
}
| plugins/packages/video_player/video_player_web/lib/video_player_web.dart/0 | {
"file_path": "plugins/packages/video_player/video_player_web/lib/video_player_web.dart",
"repo_id": "plugins",
"token_count": 1513
} | 1,246 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_test/flutter_test.dart';
import 'package:mockito/annotations.dart';
import 'package:mockito/mockito.dart';
import 'package:webview_flutter/webview_flutter.dart';
import 'package:webview_flutter_platform_interface/webview_flutter_platform_interface.dart';
import 'webview_cookie_manager_test.mocks.dart';
@GenerateMocks(<Type>[PlatformWebViewCookieManager])
void main() {
group('WebViewCookieManager', () {
test('clearCookies', () async {
final MockPlatformWebViewCookieManager mockPlatformWebViewCookieManager =
MockPlatformWebViewCookieManager();
when(mockPlatformWebViewCookieManager.clearCookies()).thenAnswer(
(_) => Future<bool>.value(false),
);
final WebViewCookieManager cookieManager =
WebViewCookieManager.fromPlatform(
mockPlatformWebViewCookieManager,
);
await expectLater(cookieManager.clearCookies(), completion(false));
});
test('setCookie', () async {
final MockPlatformWebViewCookieManager mockPlatformWebViewCookieManager =
MockPlatformWebViewCookieManager();
final WebViewCookieManager cookieManager =
WebViewCookieManager.fromPlatform(
mockPlatformWebViewCookieManager,
);
const WebViewCookie cookie = WebViewCookie(
name: 'name',
value: 'value',
domain: 'domain',
);
await cookieManager.setCookie(cookie);
final WebViewCookie capturedCookie = verify(
mockPlatformWebViewCookieManager.setCookie(captureAny),
).captured.single as WebViewCookie;
expect(capturedCookie, cookie);
});
});
}
| plugins/packages/webview_flutter/webview_flutter/test/webview_cookie_manager_test.dart/0 | {
"file_path": "plugins/packages/webview_flutter/webview_flutter/test/webview_cookie_manager_test.dart",
"repo_id": "plugins",
"token_count": 653
} | 1,247 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.webviewflutter;
import android.os.Build;
import android.webkit.WebChromeClient;
import androidx.annotation.RequiresApi;
import io.flutter.plugin.common.BinaryMessenger;
import java.util.Arrays;
/**
* Flutter Api implementation for {@link android.webkit.WebChromeClient.FileChooserParams}.
*
* <p>Passes arguments of callbacks methods from a {@link
* android.webkit.WebChromeClient.FileChooserParams} to Dart.
*/
@RequiresApi(api = Build.VERSION_CODES.LOLLIPOP)
public class FileChooserParamsFlutterApiImpl
extends GeneratedAndroidWebView.FileChooserParamsFlutterApi {
private final InstanceManager instanceManager;
/**
* Creates a Flutter api that sends messages to Dart.
*
* @param binaryMessenger handles sending messages to Dart
* @param instanceManager maintains instances stored to communicate with Dart objects
*/
public FileChooserParamsFlutterApiImpl(
BinaryMessenger binaryMessenger, InstanceManager instanceManager) {
super(binaryMessenger);
this.instanceManager = instanceManager;
}
private static GeneratedAndroidWebView.FileChooserModeEnumData toFileChooserEnumData(int mode) {
final GeneratedAndroidWebView.FileChooserModeEnumData.Builder builder =
new GeneratedAndroidWebView.FileChooserModeEnumData.Builder();
switch (mode) {
case WebChromeClient.FileChooserParams.MODE_OPEN:
builder.setValue(GeneratedAndroidWebView.FileChooserMode.OPEN);
break;
case WebChromeClient.FileChooserParams.MODE_OPEN_MULTIPLE:
builder.setValue(GeneratedAndroidWebView.FileChooserMode.OPEN_MULTIPLE);
break;
case WebChromeClient.FileChooserParams.MODE_SAVE:
builder.setValue(GeneratedAndroidWebView.FileChooserMode.SAVE);
break;
default:
throw new IllegalArgumentException(String.format("Unsupported FileChooserMode: %d", mode));
}
return builder.build();
}
/**
* Stores the FileChooserParams instance and notifies Dart to create a new FileChooserParams
* instance that is attached to this one.
*
* @return the instanceId of the stored instance
*/
public long create(WebChromeClient.FileChooserParams instance, Reply<Void> callback) {
final long instanceId = instanceManager.addHostCreatedInstance(instance);
create(
instanceId,
instance.isCaptureEnabled(),
Arrays.asList(instance.getAcceptTypes()),
toFileChooserEnumData(instance.getMode()),
instance.getFilenameHint(),
callback);
return instanceId;
}
}
| plugins/packages/webview_flutter/webview_flutter_android/android/src/main/java/io/flutter/plugins/webviewflutter/FileChooserParamsFlutterApiImpl.java/0 | {
"file_path": "plugins/packages/webview_flutter/webview_flutter_android/android/src/main/java/io/flutter/plugins/webviewflutter/FileChooserParamsFlutterApiImpl.java",
"repo_id": "plugins",
"token_count": 913
} | 1,248 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.webviewflutter;
import android.annotation.SuppressLint;
import android.os.Build;
import android.webkit.WebResourceError;
import android.webkit.WebResourceRequest;
import android.webkit.WebView;
import android.webkit.WebViewClient;
import androidx.annotation.RequiresApi;
import androidx.webkit.WebResourceErrorCompat;
import io.flutter.plugin.common.BinaryMessenger;
import io.flutter.plugins.webviewflutter.GeneratedAndroidWebView.WebViewClientFlutterApi;
import java.util.HashMap;
/**
* Flutter Api implementation for {@link WebViewClient}.
*
* <p>Passes arguments of callbacks methods from a {@link WebViewClient} to Dart.
*/
public class WebViewClientFlutterApiImpl extends WebViewClientFlutterApi {
private final InstanceManager instanceManager;
@RequiresApi(api = Build.VERSION_CODES.M)
static GeneratedAndroidWebView.WebResourceErrorData createWebResourceErrorData(
WebResourceError error) {
return new GeneratedAndroidWebView.WebResourceErrorData.Builder()
.setErrorCode((long) error.getErrorCode())
.setDescription(error.getDescription().toString())
.build();
}
@SuppressLint("RequiresFeature")
static GeneratedAndroidWebView.WebResourceErrorData createWebResourceErrorData(
WebResourceErrorCompat error) {
return new GeneratedAndroidWebView.WebResourceErrorData.Builder()
.setErrorCode((long) error.getErrorCode())
.setDescription(error.getDescription().toString())
.build();
}
@RequiresApi(api = Build.VERSION_CODES.LOLLIPOP)
static GeneratedAndroidWebView.WebResourceRequestData createWebResourceRequestData(
WebResourceRequest request) {
final GeneratedAndroidWebView.WebResourceRequestData.Builder requestData =
new GeneratedAndroidWebView.WebResourceRequestData.Builder()
.setUrl(request.getUrl().toString())
.setIsForMainFrame(request.isForMainFrame())
.setHasGesture(request.hasGesture())
.setMethod(request.getMethod())
.setRequestHeaders(
request.getRequestHeaders() != null
? request.getRequestHeaders()
: new HashMap<>());
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.N) {
requestData.setIsRedirect(request.isRedirect());
}
return requestData.build();
}
/**
* Creates a Flutter api that sends messages to Dart.
*
* @param binaryMessenger handles sending messages to Dart
* @param instanceManager maintains instances stored to communicate with Dart objects
*/
public WebViewClientFlutterApiImpl(
BinaryMessenger binaryMessenger, InstanceManager instanceManager) {
super(binaryMessenger);
this.instanceManager = instanceManager;
}
/** Passes arguments from {@link WebViewClient#onPageStarted} to Dart. */
public void onPageStarted(
WebViewClient webViewClient, WebView webView, String urlArg, Reply<Void> callback) {
final Long webViewIdentifier = instanceManager.getIdentifierForStrongReference(webView);
if (webViewIdentifier == null) {
throw new IllegalStateException("Could not find identifier for WebView.");
}
onPageStarted(getIdentifierForClient(webViewClient), webViewIdentifier, urlArg, callback);
}
/** Passes arguments from {@link WebViewClient#onPageFinished} to Dart. */
public void onPageFinished(
WebViewClient webViewClient, WebView webView, String urlArg, Reply<Void> callback) {
final Long webViewIdentifier = instanceManager.getIdentifierForStrongReference(webView);
if (webViewIdentifier == null) {
throw new IllegalStateException("Could not find identifier for WebView.");
}
onPageFinished(getIdentifierForClient(webViewClient), webViewIdentifier, urlArg, callback);
}
/**
* Passes arguments from {@link WebViewClient#onReceivedError(WebView, WebResourceRequest,
* WebResourceError)} to Dart.
*/
@RequiresApi(api = Build.VERSION_CODES.M)
public void onReceivedRequestError(
WebViewClient webViewClient,
WebView webView,
WebResourceRequest request,
WebResourceError error,
Reply<Void> callback) {
final Long webViewIdentifier = instanceManager.getIdentifierForStrongReference(webView);
if (webViewIdentifier == null) {
throw new IllegalStateException("Could not find identifier for WebView.");
}
onReceivedRequestError(
getIdentifierForClient(webViewClient),
webViewIdentifier,
createWebResourceRequestData(request),
createWebResourceErrorData(error),
callback);
}
/**
* Passes arguments from {@link androidx.webkit.WebViewClientCompat#onReceivedError(WebView,
* WebResourceRequest, WebResourceError)} to Dart.
*/
@RequiresApi(api = Build.VERSION_CODES.LOLLIPOP)
public void onReceivedRequestError(
WebViewClient webViewClient,
WebView webView,
WebResourceRequest request,
WebResourceErrorCompat error,
Reply<Void> callback) {
final Long webViewIdentifier = instanceManager.getIdentifierForStrongReference(webView);
if (webViewIdentifier == null) {
throw new IllegalStateException("Could not find identifier for WebView.");
}
onReceivedRequestError(
getIdentifierForClient(webViewClient),
webViewIdentifier,
createWebResourceRequestData(request),
createWebResourceErrorData(error),
callback);
}
/**
* Passes arguments from {@link WebViewClient#onReceivedError(WebView, int, String, String)} to
* Dart.
*/
public void onReceivedError(
WebViewClient webViewClient,
WebView webView,
Long errorCodeArg,
String descriptionArg,
String failingUrlArg,
Reply<Void> callback) {
final Long webViewIdentifier = instanceManager.getIdentifierForStrongReference(webView);
if (webViewIdentifier == null) {
throw new IllegalStateException("Could not find identifier for WebView.");
}
onReceivedError(
getIdentifierForClient(webViewClient),
webViewIdentifier,
errorCodeArg,
descriptionArg,
failingUrlArg,
callback);
}
/**
* Passes arguments from {@link WebViewClient#shouldOverrideUrlLoading(WebView,
* WebResourceRequest)} to Dart.
*/
@RequiresApi(api = Build.VERSION_CODES.LOLLIPOP)
public void requestLoading(
WebViewClient webViewClient,
WebView webView,
WebResourceRequest request,
Reply<Void> callback) {
final Long webViewIdentifier = instanceManager.getIdentifierForStrongReference(webView);
if (webViewIdentifier == null) {
throw new IllegalStateException("Could not find identifier for WebView.");
}
requestLoading(
getIdentifierForClient(webViewClient),
webViewIdentifier,
createWebResourceRequestData(request),
callback);
}
/**
* Passes arguments from {@link WebViewClient#shouldOverrideUrlLoading(WebView, String)} to Dart.
*/
public void urlLoading(
WebViewClient webViewClient, WebView webView, String urlArg, Reply<Void> callback) {
final Long webViewIdentifier = instanceManager.getIdentifierForStrongReference(webView);
if (webViewIdentifier == null) {
throw new IllegalStateException("Could not find identifier for WebView.");
}
urlLoading(getIdentifierForClient(webViewClient), webViewIdentifier, urlArg, callback);
}
private long getIdentifierForClient(WebViewClient webViewClient) {
final Long identifier = instanceManager.getIdentifierForStrongReference(webViewClient);
if (identifier == null) {
throw new IllegalStateException("Could not find identifier for WebViewClient.");
}
return identifier;
}
}
| plugins/packages/webview_flutter/webview_flutter_android/android/src/main/java/io/flutter/plugins/webviewflutter/WebViewClientFlutterApiImpl.java/0 | {
"file_path": "plugins/packages/webview_flutter/webview_flutter_android/android/src/main/java/io/flutter/plugins/webviewflutter/WebViewClientFlutterApiImpl.java",
"repo_id": "plugins",
"token_count": 2653
} | 1,249 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.