text
stringlengths 6
13.6M
| id
stringlengths 13
176
| metadata
dict | __index_level_0__
int64 0
1.69k
|
---|---|---|---|
/*
* Copyright 2018 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.project;
import com.intellij.ide.util.importProject.ModuleDescriptor;
import com.intellij.ide.util.importProject.ProjectDescriptor;
import com.intellij.ide.util.projectWizard.importSources.DetectedProjectRoot;
import com.intellij.ide.util.projectWizard.importSources.ProjectFromSourcesBuilder;
import com.intellij.ide.util.projectWizard.importSources.ProjectStructureDetector;
import com.intellij.openapi.application.ApplicationManager;
import com.intellij.openapi.diagnostic.Logger;
import com.intellij.openapi.module.Module;
import com.intellij.openapi.module.ModuleManager;
import com.intellij.openapi.project.DumbService;
import com.intellij.openapi.project.Project;
import com.intellij.openapi.project.ProjectManager;
import com.intellij.openapi.project.ProjectManagerListener;
import com.intellij.openapi.roots.ModuleRootManager;
import com.intellij.openapi.startup.StartupManager;
import com.intellij.openapi.vfs.LocalFileSystem;
import com.intellij.openapi.vfs.VfsUtil;
import com.intellij.openapi.vfs.VirtualFile;
import com.intellij.util.messages.MessageBusConnection;
import io.flutter.module.FlutterModuleBuilder;
import io.flutter.pub.PubRoot;
import io.flutter.utils.FlutterModuleUtils;
import org.jetbrains.annotations.NotNull;
import java.io.File;
import java.util.*;
public class FlutterProjectStructureDetector extends ProjectStructureDetector {
private static final Logger LOG = Logger.getInstance(ProjectStructureDetector.class);
@NotNull
@Override
public DirectoryProcessingResult detectRoots(@NotNull File parent,
@SuppressWarnings("NullableProblems") @NotNull File[] children,
@NotNull File base,
@NotNull List<DetectedProjectRoot> result) {
final VirtualFile dir = VfsUtil.findFileByIoFile(parent, false);
if (dir != null) {
final PubRoot pubRoot = PubRoot.forDirectory(dir);
if (pubRoot != null) {
if (pubRoot.declaresFlutter()) {
result.add(new FlutterProjectRoot(parent));
}
else {
// TODO(pq): consider pushing pure dart project detection down into the Dart Plugin.
result.add(new DartProjectRoot(parent));
}
return DirectoryProcessingResult.SKIP_CHILDREN;
}
}
return DirectoryProcessingResult.PROCESS_CHILDREN;
}
@Override
public String getDetectorId() {
return "Flutter";
}
@Override
public void setupProjectStructure(@NotNull Collection<DetectedProjectRoot> roots,
@NotNull ProjectDescriptor projectDescriptor,
@NotNull ProjectFromSourcesBuilder builder) {
final List<ModuleDescriptor> modules = new ArrayList<>();
for (DetectedProjectRoot root : roots) {
if (root != null) {
//noinspection ConstantConditions
modules.add(new ModuleDescriptor(root.getDirectory(), FlutterModuleUtils.getFlutterModuleType(), Collections.emptyList()));
}
}
projectDescriptor.setModules(modules);
builder.setupModulesByContentRoots(projectDescriptor, roots);
String name = builder.getContext().getProjectName();
if (name != null) {
scheduleAndroidModuleAddition(name, modules, 0);
}
}
private void scheduleAndroidModuleAddition(@NotNull String projectName, @NotNull List<ModuleDescriptor> modules, int tries) {
//noinspection ConstantConditions
final MessageBusConnection[] connection = {ApplicationManager.getApplication().getMessageBus().connect()};
scheduleDisconnectIfCancelled(connection);
//noinspection ConstantConditions
connection[0].subscribe(ProjectManager.TOPIC, new ProjectManagerListener() {
//See https://plugins.jetbrains.com/docs/intellij/plugin-components.html#comintellijpoststartupactivity
// for notice and documentation on the deprecation intentions of
// Components from JetBrains.
//
// Migration forward has different directions before and after
// 2023.1, if we can, it would be prudent to wait until we are
// only supporting this major platform as a minimum version.
//
// https://github.com/flutter/flutter-intellij/issues/6953
@Override
public void projectOpened(@NotNull Project project) {
if (connection[0] != null) {
connection[0].disconnect();
connection[0] = null;
}
if (!projectName.equals(project.getName())) {
// This can happen if you have selected project roots in the import wizard then cancel the import,
// and then import a project with a different name, before the scheduled disconnect runs.
return;
}
//noinspection ConstantConditions
StartupManager.getInstance(project).runAfterOpened(() -> {
//noinspection ConstantConditions
DumbService.getInstance(project).smartInvokeLater(() -> {
for (ModuleDescriptor module : modules) {
assert module != null;
Set<File> roots = module.getContentRoots();
String moduleName = module.getName();
if (roots == null || roots.size() != 1 || moduleName == null) continue;
File root = roots.iterator().next();
assert root != null;
if (!projectHasContentRoot(project, root)) continue;
String imlName = moduleName + "_android.iml";
File moduleDir = null;
if (new File(root, imlName).exists()) {
moduleDir = root;
}
else {
for (String name : new String[]{"android", ".android"}) {
File dir = new File(root, name);
if (dir.exists() && new File(dir, imlName).exists()) {
moduleDir = dir;
break;
}
}
}
if (moduleDir == null) continue;
try {
// Searching for a module by name and skipping the next line if found,
// will not always eliminate the exception caught here.
// Specifically, if the project had previously been opened and the caches were not cleared.
//noinspection ConstantConditions
FlutterModuleBuilder.addAndroidModule(project, null, moduleDir.getPath(), module.getName(), true);
}
catch (IllegalStateException ignored) {
}
// Check for a plugin example module.
File example = new File(root, "example");
if (example.exists()) {
File android = new File(example, "android");
File exampleFile;
if (android.exists() && (exampleFile = new File(android, moduleName + "_example_android.iml")).exists()) {
try {
//noinspection ConstantConditions
FlutterModuleBuilder.addAndroidModuleFromFile(project, null,
LocalFileSystem.getInstance().findFileByIoFile(exampleFile));
}
catch (IllegalStateException ignored) {
}
}
}
}
});
});
}
});
}
@SuppressWarnings("ConstantConditions")
private static void scheduleDisconnectIfCancelled(MessageBusConnection[] connection) {
// If the import was cancelled the subscription will never be removed.
ApplicationManager.getApplication().executeOnPooledThread(() -> {
Project project = ProjectManager.getInstance().getDefaultProject();
try {
Thread.sleep(300000L); // Allow five minutes to complete the project import wizard.
}
catch (InterruptedException ignored) {
}
if (connection[0] != null) {
connection[0].disconnect();
connection[0] = null;
}
});
}
@SuppressWarnings("ConstantConditions")
private static boolean projectHasContentRoot(@NotNull Project project, @NotNull File root) {
// Verify that the project has the given content root. If the import was cancelled and restarted
// for the same project, but a content root was not selected the second time, then it might be absent.
VirtualFile virtualFile = LocalFileSystem.getInstance().findFileByIoFile(root);
for (Module module : ModuleManager.getInstance(project).getModules()) {
for (VirtualFile file : ModuleRootManager.getInstance(module).getContentRoots()) {
if (file != null && file.equals(virtualFile)) {
return true;
}
}
}
return false;
}
private static class FlutterProjectRoot extends DetectedProjectRoot {
public FlutterProjectRoot(@NotNull File directory) {
super(directory);
}
@NotNull
@Override
public String getRootTypeName() {
return "Flutter";
}
}
private static class DartProjectRoot extends DetectedProjectRoot {
public DartProjectRoot(@NotNull File directory) {
super(directory);
}
@NotNull
@Override
public String getRootTypeName() {
return "Dart";
}
}
}
| flutter-intellij/flutter-idea/src/io/flutter/project/FlutterProjectStructureDetector.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/project/FlutterProjectStructureDetector.java",
"repo_id": "flutter-intellij",
"token_count": 3735
} | 451 |
/*
* Copyright 2016 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.run;
import com.intellij.execution.actions.ConfigurationContext;
import com.intellij.execution.actions.ConfigurationFromContext;
import com.intellij.execution.actions.RunConfigurationProducer;
import com.intellij.openapi.roots.ProjectFileIndex;
import com.intellij.openapi.util.Ref;
import com.intellij.openapi.vfs.VirtualFile;
import com.intellij.psi.PsiElement;
import com.intellij.psi.util.PsiTreeUtil;
import com.jetbrains.lang.dart.psi.DartFile;
import com.jetbrains.lang.dart.psi.DartImportStatement;
import com.jetbrains.lang.dart.util.DartResolveUtil;
import io.flutter.FlutterUtils;
import io.flutter.dart.DartPlugin;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.util.Arrays;
import java.util.stream.Stream;
/**
* Determines when we can run a Dart file as a Flutter app.
* <p>
* (For example, when right-clicking on main.dart in the Project view.)
*/
public class FlutterRunConfigurationProducer extends RunConfigurationProducer<SdkRunConfig> {
public FlutterRunConfigurationProducer() {
super(FlutterRunConfigurationType.getInstance());
}
/**
* If the current file contains the main method for a Flutter app, updates the FutterRunConfiguration
* and sets its corresponding source location.
* <p>
* Returns false if it wasn't a match.
*/
@Override
protected boolean setupConfigurationFromContext(final @NotNull SdkRunConfig config,
final @NotNull ConfigurationContext context,
final @NotNull Ref<PsiElement> sourceElement) {
final VirtualFile main = getFlutterEntryFile(context, true, true);
if (main == null) return false;
config.setFields(new SdkFields(main));
config.setGeneratedName();
final PsiElement elt = sourceElement.get();
if (elt != null) {
sourceElement.set(elt.getContainingFile());
}
return true;
}
/**
* Returns true if an existing SdkRunConfig points to the current Dart file.
*/
@Override
public boolean isConfigurationFromContext(final @NotNull SdkRunConfig configuration,
final @NotNull ConfigurationContext context) {
return hasDartFile(context, configuration.getFields().getFilePath());
}
/**
* Returns true if Flutter's run configuration should take priority over another one that
* applies to the same source file.
*/
@Override
public boolean shouldReplace(@NotNull ConfigurationFromContext self, @NotNull ConfigurationFromContext other) {
// Prefer Flutter runner to plain Dart runner for Flutter code.
return DartPlugin.isDartRunConfiguration(other.getConfigurationType());
}
/**
* Returns the file containing a Flutter app's main() function, or null if not a match.
*/
@Nullable
public static VirtualFile getFlutterEntryFile(final @NotNull ConfigurationContext context,
boolean requireFlutterImport,
boolean omitTests) {
final DartFile dart = getDartFile(context);
return getFlutterEntryFile(dart, requireFlutterImport, omitTests);
}
/**
* Returns the corresponding virtual file containing a Flutter app's main() function, or null if not a match.
*/
@Nullable
public static VirtualFile getFlutterEntryFile(final @Nullable DartFile dart, boolean requireFlutterImport, boolean omitTests) {
if (dart == null) return null;
if (DartResolveUtil.getMainFunction(dart) == null) return null;
if (requireFlutterImport && findImportUrls(dart).noneMatch((url) -> url.startsWith("package:flutter/"))) {
return null;
}
if (omitTests) {
if (findImportUrls(dart).anyMatch((url) -> url.startsWith("package:flutter_test/"))) {
return null;
}
if (findImportUrls(dart).anyMatch((url) -> url.startsWith("package:test/"))) {
return null;
}
}
final VirtualFile virtual = DartResolveUtil.getRealVirtualFile(dart);
if (virtual == null) return null;
if (!ProjectFileIndex.getInstance(dart.getProject()).isInContent(virtual)) {
return null;
}
return virtual;
}
/**
* Returns true if the context points to the given file and it's a Dart file.
*/
public static boolean hasDartFile(@NotNull ConfigurationContext context, String dartPath) {
final DartFile dart = getDartFile(context);
if (dart == null) {
return false;
}
final VirtualFile virtual = DartResolveUtil.getRealVirtualFile(dart);
return virtual != null && virtual.getPath().equals(dartPath);
}
/**
* Returns the Dart file at the current location, or null if not a match.
*/
public static @Nullable
DartFile getDartFile(final @NotNull ConfigurationContext context) {
return FlutterUtils.getDartFile(context.getPsiLocation());
}
/**
* Returns the import URL's in a Dart file.
*/
private static @NotNull
Stream<String> findImportUrls(@NotNull DartFile file) {
final DartImportStatement[] imports = PsiTreeUtil.getChildrenOfType(file, DartImportStatement.class);
if (imports == null) return Stream.empty();
return Arrays.stream(imports).map(DartImportStatement::getUriString);
}
}
| flutter-intellij/flutter-idea/src/io/flutter/run/FlutterRunConfigurationProducer.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/run/FlutterRunConfigurationProducer.java",
"repo_id": "flutter-intellij",
"token_count": 1909
} | 452 |
<?xml version="1.0" encoding="UTF-8"?>
<form xmlns="http://www.intellij.com/uidesigner/form/" version="1" bind-to-class="io.flutter.run.bazel.FlutterBazelConfigurationEditorForm">
<grid id="27dc6" binding="myMainPanel" default-binding="true" layout-manager="GridLayoutManager" row-count="9" column-count="3" same-size-horizontally="false" same-size-vertically="false" hgap="-1" vgap="-1">
<margin top="0" left="0" bottom="0" right="0"/>
<constraints>
<xy x="20" y="20" width="916" height="336"/>
</constraints>
<properties/>
<border type="none"/>
<children>
<vspacer id="3f5de">
<constraints>
<grid row="8" column="0" row-span="1" col-span="2" vsize-policy="6" hsize-policy="1" anchor="0" fill="2" indent="0" use-parent-layout="false"/>
</constraints>
</vspacer>
<component id="7b562" class="javax.swing.JLabel">
<constraints>
<grid row="6" column="0" row-span="1" col-span="2" vsize-policy="0" hsize-policy="0" anchor="8" fill="0" indent="0" use-parent-layout="false"/>
</constraints>
<properties>
<labelFor value="ada9e"/>
<text value="Additional &args:"/>
</properties>
</component>
<component id="7f926" class="javax.swing.JLabel">
<constraints>
<grid row="7" column="2" row-span="1" col-span="1" vsize-policy="0" hsize-policy="0" anchor="8" fill="0" indent="0" use-parent-layout="false"/>
</constraints>
<properties>
<enabled value="false"/>
<text value="Additional arguments to pass to the Flutter runner (e.g. --enable-software-rendering)."/>
</properties>
</component>
<component id="ada9e" class="javax.swing.JTextField" binding="myAdditionalArgs">
<constraints>
<grid row="6" column="2" row-span="1" col-span="1" vsize-policy="0" hsize-policy="6" anchor="0" fill="1" indent="0" use-parent-layout="false"/>
</constraints>
<properties/>
</component>
<component id="5a54b" class="javax.swing.JCheckBox" binding="myEnableReleaseModeCheckBox" default-binding="true">
<constraints>
<grid row="2" column="2" row-span="1" col-span="1" vsize-policy="0" hsize-policy="3" anchor="8" fill="0" indent="0" use-parent-layout="false"/>
</constraints>
<properties>
<enabled value="true"/>
<selected value="false"/>
<text value="Enable release mode, --define flutter_build_mode=release"/>
</properties>
</component>
<component id="f7b9" class="javax.swing.JLabel">
<constraints>
<grid row="2" column="0" row-span="1" col-span="2" vsize-policy="0" hsize-policy="0" anchor="8" fill="0" indent="0" use-parent-layout="false"/>
</constraints>
<properties>
<text value="&Release mode:"/>
</properties>
</component>
<component id="66407" class="javax.swing.JLabel">
<constraints>
<grid row="3" column="2" row-span="1" col-span="1" vsize-policy="0" hsize-policy="0" anchor="8" fill="0" indent="0" use-parent-layout="false"/>
</constraints>
<properties>
<enabled value="false"/>
<text value="Release mode does not support debugging, and a physical device will be required when launching."/>
</properties>
</component>
<component id="c77fb" class="javax.swing.JLabel">
<constraints>
<grid row="4" column="0" row-span="1" col-span="2" vsize-policy="0" hsize-policy="0" anchor="8" fill="0" indent="0" use-parent-layout="false"/>
</constraints>
<properties>
<labelFor value="ada9e"/>
<text value="Bazel args"/>
</properties>
</component>
<component id="a8455" class="javax.swing.JLabel">
<constraints>
<grid row="5" column="2" row-span="1" col-span="1" vsize-policy="0" hsize-policy="0" anchor="8" fill="0" indent="0" use-parent-layout="false"/>
</constraints>
<properties>
<enabled value="false"/>
<text value="Additional arguments to pass to the Bazel runtime (e.g. --define=release_channel=beta3)"/>
</properties>
</component>
<component id="9e12e" class="javax.swing.JTextField" binding="myBazelArgs">
<constraints>
<grid row="4" column="2" row-span="1" col-span="1" vsize-policy="0" hsize-policy="6" anchor="0" fill="1" indent="0" use-parent-layout="false"/>
</constraints>
<properties/>
</component>
<component id="1b4c4" class="javax.swing.JLabel">
<constraints>
<grid row="1" column="2" row-span="1" col-span="1" vsize-policy="0" hsize-policy="0" anchor="8" fill="0" indent="0" use-parent-layout="false"/>
</constraints>
<properties>
<enabled value="false"/>
<text value="The Bazel target (e.g. //a/b/c:name) or entrypoint for the application (e.g. main.dart)"/>
</properties>
</component>
<component id="bda6b" class="javax.swing.JLabel">
<constraints>
<grid row="0" column="0" row-span="1" col-span="1" vsize-policy="0" hsize-policy="0" anchor="8" fill="0" indent="0" use-parent-layout="false"/>
</constraints>
<properties>
<text value="Target or Dart entrypoint:"/>
<verifyInputWhenFocusTarget value="false"/>
</properties>
</component>
<component id="1b95c" class="com.intellij.openapi.ui.TextFieldWithBrowseButton" binding="myTarget">
<constraints>
<grid row="0" column="2" row-span="1" col-span="1" vsize-policy="3" hsize-policy="3" anchor="0" fill="1" indent="0" use-parent-layout="false"/>
</constraints>
<properties>
<focusable value="true"/>
</properties>
</component>
</children>
</grid>
</form>
| flutter-intellij/flutter-idea/src/io/flutter/run/bazel/FlutterBazelConfigurationEditorForm.form/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/run/bazel/FlutterBazelConfigurationEditorForm.form",
"repo_id": "flutter-intellij",
"token_count": 2608
} | 453 |
/*
* Copyright 2019 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.run.common;
import com.intellij.execution.Executor;
import com.intellij.execution.configurations.RunConfiguration;
import com.intellij.execution.testframework.TestConsoleProperties;
import com.intellij.execution.testframework.actions.AbstractRerunFailedTestsAction;
import com.intellij.execution.testframework.sm.SMCustomMessagesParsing;
import com.intellij.execution.testframework.sm.runner.OutputToGeneralTestEventsConverter;
import com.intellij.execution.testframework.sm.runner.SMTRunnerConsoleProperties;
import com.intellij.execution.testframework.sm.runner.SMTestLocator;
import com.intellij.execution.ui.ConsoleView;
import com.jetbrains.lang.dart.util.DartUrlResolver;
import io.flutter.run.bazelTest.BazelTestConfig;
import io.flutter.run.test.FlutterTestEventsConverter;
import io.flutter.run.test.FlutterTestLocationProvider;
import io.flutter.run.test.TestConfig;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
/**
* Configuration for the test console.
* <p>
* In particular, configures how it parses test events and handles the re-run action.
*/
public class ConsoleProps extends SMTRunnerConsoleProperties implements SMCustomMessagesParsing {
/**
* Name of the {@code testFrameworkName}passed to the {@link ConsoleProps} constructor for Pub-based consoles.
*/
public static String pubFrameworkName = "FlutterTestRunner";
/**
* Name of the {@code testFrameworkName}passed to the {@link ConsoleProps} constructor for Bazel-based consoles..
*/
public static String bazelFrameworkName = "FlutterBazelTestRunner";
@NotNull
private final DartUrlResolver resolver;
private ConsoleProps(@NotNull RunConfiguration config,
@NotNull Executor exec,
@NotNull DartUrlResolver resolver,
String testFrameworkName) {
super(config, "FlutterTestRunner", exec);
this.resolver = resolver;
setUsePredefinedMessageFilter(false);
setIdBasedTestTree(true);
}
public static ConsoleProps forPub(@NotNull TestConfig config, @NotNull Executor exec, @NotNull DartUrlResolver resolver) {
return new ConsoleProps(config, exec, resolver, pubFrameworkName);
}
public static ConsoleProps forBazel(@NotNull BazelTestConfig config, @NotNull Executor exec, @NotNull DartUrlResolver resolver) {
return new ConsoleProps(config, exec, resolver, bazelFrameworkName);
}
@Nullable
@Override
public SMTestLocator getTestLocator() {
return FlutterTestLocationProvider.INSTANCE;
}
@Override
public OutputToGeneralTestEventsConverter createTestEventsConverter(@NotNull String testFrameworkName,
@NotNull TestConsoleProperties props) {
return new FlutterTestEventsConverter(testFrameworkName, props, resolver);
}
@Nullable
@Override
public AbstractRerunFailedTestsAction createRerunFailedTestsAction(ConsoleView consoleView) {
// TODO(github.com/flutter/flutter-intellij/issues/3504): Implement this.
return null;
}
}
| flutter-intellij/flutter-idea/src/io/flutter/run/common/ConsoleProps.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/run/common/ConsoleProps.java",
"repo_id": "flutter-intellij",
"token_count": 1104
} | 454 |
/*
* Copyright 2017 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.run.daemon;
import com.google.common.base.Joiner;
import com.google.common.base.Objects;
import com.google.common.collect.ImmutableList;
import com.intellij.execution.ExecutionException;
import com.intellij.execution.configurations.GeneralCommandLine;
import com.intellij.execution.process.ProcessHandler;
import com.intellij.openapi.application.ApplicationManager;
import com.intellij.openapi.application.ModalityState;
import com.intellij.openapi.diagnostic.Logger;
import com.intellij.openapi.project.Project;
import com.intellij.openapi.ui.DialogWrapper;
import com.intellij.openapi.ui.Messages;
import com.intellij.openapi.util.SystemInfo;
import com.intellij.openapi.util.io.FileUtil;
import io.flutter.FlutterMessages;
import io.flutter.FlutterUtils;
import io.flutter.android.IntelliJAndroidSdk;
import io.flutter.bazel.Workspace;
import io.flutter.bazel.WorkspaceCache;
import io.flutter.run.FlutterDevice;
import io.flutter.sdk.FlutterSdk;
import io.flutter.sdk.FlutterSdkUtil;
import io.flutter.utils.FlutterModuleUtils;
import io.flutter.utils.MostlySilentColoredProcessHandler;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import javax.swing.*;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
import java.util.concurrent.*;
import java.util.concurrent.atomic.AtomicBoolean;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.concurrent.atomic.AtomicReference;
import java.util.function.Consumer;
import java.util.function.Supplier;
import java.util.stream.Stream;
/**
* A process running 'flutter daemon' to watch for devices.
*/
class DeviceDaemon {
private static final AtomicInteger nextDaemonId = new AtomicInteger();
/**
* Attempt to start the daemon this many times before showing the user a warning that the daemon is having trouble starting up.
*/
private static final int RESTART_ATTEMPTS_BEFORE_WARNING = 1;
/**
* A unique id used to log device daemon actions.
*/
private final int id;
/**
* The command used to start this daemon.
*/
@NotNull private final Command command;
@NotNull private final ProcessHandler process;
@NotNull private final Listener listener;
@NotNull private final AtomicReference<ImmutableList<FlutterDevice>> devices;
private DeviceDaemon(int id,
@NotNull Command command, @NotNull ProcessHandler process, @NotNull Listener listener,
@NotNull AtomicReference<ImmutableList<FlutterDevice>> devices) {
this.id = id;
this.command = command;
this.process = process;
this.listener = listener;
this.devices = devices;
listener.running.set(true);
}
/**
* Returns true if the process is still running.
*/
boolean isRunning() {
return !process.isProcessTerminating() && !process.isProcessTerminated();
}
/**
* Returns the current devices.
* <p>
* <p>This is calculated based on add and remove events seen since the process started.
*/
ImmutableList<FlutterDevice> getDevices() {
return devices.get();
}
/**
* Returns true if the daemon should be restarted.
*
* @param next the command that should be running now.
*/
boolean needRestart(@NotNull Command next) {
return !isRunning() || !command.equals(next);
}
/**
* Kills the process. (Normal shutdown.)
*/
void shutdown() {
if (!process.isProcessTerminated()) {
LOG.info("shutting down Flutter device daemon #" + id + ": " + command);
}
listener.running.set(false);
process.destroyProcess();
}
/**
* Returns the appropriate command to start the device daemon, if any.
* <p>
* A null means the device daemon should be shut down.
*/
@Nullable
static Command chooseCommand(@NotNull final Project project) {
if (!usesFlutter(project)) {
return null;
}
final String androidHome = IntelliJAndroidSdk.chooseAndroidHome(project, false);
// See if the Bazel workspace provides a script.
final Workspace workspace = WorkspaceCache.getInstance(project).get();
if (workspace != null) {
final String script = workspace.getDaemonScript();
if (script != null) {
return new Command(workspace.getRoot().getPath(), script, ImmutableList.of(), androidHome);
}
}
// Otherwise, use the Flutter SDK.
final FlutterSdk sdk = FlutterSdk.getFlutterSdk(project);
if (sdk == null) {
return null;
}
try {
final String path = FlutterSdkUtil.pathToFlutterTool(sdk.getHomePath());
final ImmutableList<String> list;
if (FlutterUtils.isIntegrationTestingMode()) {
list = ImmutableList.of("--show-test-device", "daemon");
}
else {
list = ImmutableList.of("daemon");
}
return new Command(sdk.getHomePath(), path, list, androidHome);
}
catch (ExecutionException e) {
FlutterUtils.warn(LOG, "Unable to calculate command to watch Flutter devices", e);
return null;
}
}
private static boolean usesFlutter(@NotNull final Project project) {
return FlutterModuleUtils.isFlutterBazelProject(project) || FlutterModuleUtils.hasFlutterModule(project);
}
/**
* The command used to start the daemon.
* <p>
* <p>Comparing two Commands lets us detect configuration changes that require a restart.
*/
static class Command {
/**
* Path to working directory for running the script. Should be an absolute path.
*/
@NotNull private final String workDir;
@NotNull private final String command;
@NotNull private final ImmutableList<String> parameters;
/**
* The value of ANDROID_HOME to use when launching the command.
*/
@Nullable private final String androidHome;
private Command(@NotNull String workDir, @NotNull String command, @NotNull ImmutableList<String> parameters,
@Nullable String androidHome) {
this.workDir = workDir;
this.command = command;
this.parameters = parameters;
this.androidHome = androidHome;
}
/**
* Launches the daemon.
*
* @param isCancelled will be polled during startup to see if startup is cancelled.
* @param deviceChanged will be called whenever a device is added or removed from the returned DeviceDaemon.
* @param processStopped will be called if the process exits unexpectedly after this method returns.
*/
DeviceDaemon start(Supplier<Boolean> isCancelled,
Runnable deviceChanged,
Consumer<String> processStopped) throws ExecutionException {
final int daemonId = nextDaemonId.incrementAndGet();
//noinspection UnnecessaryToStringCall
LOG.info("starting Flutter device daemon #" + daemonId + ": " + toString());
// The mostly silent process handler reduces CPU usage of the daemon process.
final ProcessHandler process = new MostlySilentColoredProcessHandler(toCommandLine());
boolean succeeded = false;
try {
final AtomicReference<ImmutableList<FlutterDevice>> devices = new AtomicReference<>(ImmutableList.of());
final DaemonApi api = new DaemonApi(process);
final Listener listener = new Listener(daemonId, api, devices, deviceChanged, processStopped);
api.listen(process, listener);
final Future<Void> ready = listener.connected.thenCompose((Void ignored) -> api.enableDeviceEvents());
// Block until we get a response, or are cancelled.
int attempts = 0;
while (true) {
if (isCancelled.get()) {
throw new CancellationException();
}
else if (process.isProcessTerminated()) {
final Integer exitCode = process.getExitCode();
String failureMessage = "Flutter device daemon #" + daemonId + " exited (exit code " + exitCode + ")";
if (!api.getStderrTail().isEmpty()) {
failureMessage += ", stderr: " + api.getStderrTail();
}
attempts++;
if (attempts <= DeviceDaemon.RESTART_ATTEMPTS_BEFORE_WARNING) {
LOG.warn(failureMessage);
}
else {
// IntelliJ will show a generic failure message the first time we log this error.
LOG.warn(failureMessage);
// The second time we log this error, we'll show a customized message to alert the user to the specific problem.
if (attempts == DeviceDaemon.RESTART_ATTEMPTS_BEFORE_WARNING + 1) {
// Show a message in the UI when we reach the warning threshold.
FlutterMessages.showError("Flutter device daemon", failureMessage, null);
}
else if (attempts == DeviceDaemon.RESTART_ATTEMPTS_BEFORE_WARNING + 4) {
ApplicationManager.getApplication().invokeLater(() -> new DaemonCrashReporter().show(), ModalityState.NON_MODAL);
return null;
}
}
}
try {
// Retry with a longer delay if we are encountering repeated failures of the daemon.
ready.get(attempts <= DeviceDaemon.RESTART_ATTEMPTS_BEFORE_WARNING ? 100L : 10000L * attempts, TimeUnit.MILLISECONDS);
succeeded = true;
return new DeviceDaemon(daemonId, this, process, listener, devices);
}
catch (TimeoutException e) {
// Check for cancellation and try again.
}
catch (InterruptedException e) {
throw new CancellationException();
}
catch (java.util.concurrent.ExecutionException e) {
// This is not a user facing crash - we log (and no devices will be discovered).
FlutterUtils.warn(LOG, e.getCause());
}
}
}
finally {
if (!succeeded) {
process.destroyProcess();
}
}
}
@Override
public boolean equals(Object obj) {
if (!(obj instanceof Command)) {
return false;
}
final Command other = (Command)obj;
return Objects.equal(workDir, other.workDir)
&& Objects.equal(command, other.command)
&& Objects.equal(parameters, other.parameters)
&& Objects.equal(androidHome, other.androidHome);
}
@Override
public int hashCode() {
return Objects.hashCode(workDir, command, parameters, androidHome);
}
private GeneralCommandLine toCommandLine() {
final GeneralCommandLine result = new GeneralCommandLine().withWorkDirectory(workDir);
result.setCharset(StandardCharsets.UTF_8);
result.setExePath(FileUtil.toSystemDependentName(command));
result.withEnvironment(FlutterSdkUtil.FLUTTER_HOST_ENV, (new FlutterSdkUtil()).getFlutterHostEnvValue());
if (androidHome != null) {
result.withEnvironment("ANDROID_HOME", androidHome);
}
for (String param : parameters) {
result.addParameter(param);
}
return result;
}
@Override
public String toString() {
final StringBuilder out = new StringBuilder();
out.append(command);
if (!parameters.isEmpty()) {
out.append(' ');
out.append(Joiner.on(' ').join(parameters));
}
return out.toString();
}
}
/**
* Handles events sent by the device daemon process.
* <p>
* <p>Updates the device list based on incoming events.
*/
private static class Listener implements DaemonEvent.Listener {
private final int daemonId;
private final DaemonApi api;
private final AtomicReference<ImmutableList<FlutterDevice>> devices;
private final Runnable deviceChanged;
private final Consumer<String> processStopped;
private transient final CompletableFuture<Void> connected = new CompletableFuture<>();
private final AtomicBoolean running = new AtomicBoolean(false);
Listener(int daemonId,
DaemonApi api,
AtomicReference<ImmutableList<FlutterDevice>> devices,
Runnable deviceChanged,
Consumer<String> processStopped) {
this.daemonId = daemonId;
this.api = api;
this.devices = devices;
this.deviceChanged = deviceChanged;
this.processStopped = processStopped;
}
// daemon domain
@Override
public void onDaemonConnected(DaemonEvent.DaemonConnected event) {
connected.complete(null);
}
@Override
public void onDaemonLogMessage(@NotNull DaemonEvent.DaemonLogMessage message) {
LOG.info("flutter device daemon #" + daemonId + ": " + message.message);
}
@Override
public void onDaemonShowMessage(@NotNull DaemonEvent.DaemonShowMessage event) {
if ("error".equals(event.level)) {
FlutterMessages.showError(event.title, event.message, null);
}
else if ("warning".equals(event.level)) {
FlutterMessages.showWarning(event.title, event.message, null);
}
else {
FlutterMessages.showInfo(event.title, event.message, null);
}
}
// device domain
public void onDeviceAdded(@NotNull DaemonEvent.DeviceAdded event) {
if (event.id == null) {
// We can't start a flutter app on this device if it doesn't have a device id.
FlutterUtils.warn(LOG, "Ignored an event from a Flutter process without a device id: " + event);
return;
}
final FlutterDevice newDevice = new FlutterDevice(event.id,
event.emulatorId == null
? (event.name == null ? event.id : event.name)
: (event.platformType.equals("android") ? event.emulatorId : event.name),
event.platform,
event.emulator,
event.category,
event.platformType,
event.ephemeral);
devices.updateAndGet((old) -> addDevice(old.stream(), newDevice));
deviceChanged.run();
}
public void onDeviceRemoved(@NotNull DaemonEvent.DeviceRemoved event) {
devices.updateAndGet((old) -> removeDevice(old.stream(), event.id));
deviceChanged.run();
}
@Override
public void processTerminated(int exitCode) {
if (running.get()) {
processStopped.accept(
"Daemon #" + daemonId + " exited. Exit code: " + exitCode + ". Stderr:\n" +
api.getStderrTail());
}
}
// helpers
private static ImmutableList<FlutterDevice> addDevice(Stream<FlutterDevice> old, FlutterDevice newDevice) {
final List<FlutterDevice> changed = new ArrayList<>(removeDevice(old, newDevice.deviceId()));
changed.add(newDevice);
changed.sort(Comparator.comparing(FlutterDevice::deviceName));
return ImmutableList.copyOf(changed);
}
private static ImmutableList<FlutterDevice> removeDevice(Stream<FlutterDevice> old, String idToRemove) {
return ImmutableList.copyOf(old.filter((d) -> !d.deviceId().equals(idToRemove)).iterator());
}
}
private static final Logger LOG = Logger.getInstance(DeviceDaemon.class);
// If the daemon cannot be started, display a modal dialog with hopefully helpful
// instructions on how to fix the problem. This is a big problem; we really do
// need to interupt the user.
// https://github.com/flutter/flutter-intellij/issues/5521
private static class DaemonCrashReporter extends DialogWrapper {
private JPanel myPanel;
private JTextPane myTextPane;
DaemonCrashReporter() {
super(null, false, false);
setTitle("Flutter Device Daemon Crash");
myPanel = new JPanel();
myTextPane = new JTextPane();
final String os = SystemInfo.getOsNameAndVersion();
final String link = "https://www.google.com/search?q=increase maximum file handles " + os;
Messages.installHyperlinkSupport(myTextPane);
final String message =
"<html><body><p>The Flutter device daemon cannot be started. " +
"<br>Please check your configuration and restart the IDE. " +
"<br><br>You may need to <a href=\"" + link +
"\">increase the maximum number of file handles</a>" +
"<br>available globally.</body></html>";
myTextPane.setText(message);
myPanel.add(myTextPane);
init();
//noinspection ConstantConditions
getButton(getCancelAction()).setVisible(false);
}
@Nullable
@Override
protected JComponent createCenterPanel() {
return myPanel;
}
}
}
| flutter-intellij/flutter-idea/src/io/flutter/run/daemon/DeviceDaemon.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/run/daemon/DeviceDaemon.java",
"repo_id": "flutter-intellij",
"token_count": 6501
} | 455 |
<?xml version="1.0" encoding="UTF-8"?>
<form xmlns="http://www.intellij.com/uidesigner/form/" version="1" bind-to-class="io.flutter.run.test.TestForm">
<grid id="27dc6" binding="form" layout-manager="GridLayoutManager" row-count="11" column-count="2" same-size-horizontally="false" same-size-vertically="false" hgap="-1" vgap="-1">
<margin top="0" left="0" bottom="0" right="0"/>
<constraints>
<xy x="20" y="20" width="619" height="400"/>
</constraints>
<properties/>
<border type="none"/>
<children>
<vspacer id="fff30">
<constraints>
<grid row="10" column="0" row-span="1" col-span="1" vsize-policy="6" hsize-policy="1" anchor="0" fill="2" indent="0" use-parent-layout="false"/>
</constraints>
</vspacer>
<component id="3abe7" class="com.intellij.openapi.ui.TextFieldWithBrowseButton" binding="testFile">
<constraints>
<grid row="2" column="1" row-span="1" col-span="1" vsize-policy="0" hsize-policy="6" anchor="0" fill="1" indent="0" use-parent-layout="false"/>
</constraints>
<properties/>
</component>
<component id="e1221" class="javax.swing.JLabel" binding="testFileLabel">
<constraints>
<grid row="2" column="0" row-span="1" col-span="1" vsize-policy="0" hsize-policy="0" anchor="8" fill="0" indent="0" use-parent-layout="false"/>
</constraints>
<properties>
<text value="Test &file:"/>
</properties>
</component>
<component id="a65c8" class="javax.swing.JComboBox" binding="scope">
<constraints>
<grid row="0" column="1" row-span="1" col-span="1" vsize-policy="0" hsize-policy="2" anchor="8" fill="0" indent="0" use-parent-layout="false">
<preferred-size width="150" height="-1"/>
</grid>
</constraints>
<properties/>
</component>
<component id="ec603" class="javax.swing.JLabel">
<constraints>
<grid row="0" column="0" row-span="1" col-span="1" vsize-policy="0" hsize-policy="0" anchor="8" fill="0" indent="0" use-parent-layout="false">
<preferred-size width="110" height="-1"/>
</grid>
</constraints>
<properties>
<labelFor value="a65c8"/>
<text value="Test &scope:"/>
</properties>
</component>
<component id="21d5" class="javax.swing.JLabel" binding="testDirLabel">
<constraints>
<grid row="4" column="0" row-span="1" col-span="1" vsize-policy="0" hsize-policy="0" anchor="8" fill="0" indent="0" use-parent-layout="false"/>
</constraints>
<properties>
<text value="Test &directory:"/>
</properties>
</component>
<component id="4fccd" class="com.intellij.openapi.ui.TextFieldWithBrowseButton" binding="testDir">
<constraints>
<grid row="4" column="1" row-span="1" col-span="1" vsize-policy="0" hsize-policy="6" anchor="0" fill="1" indent="0" use-parent-layout="false"/>
</constraints>
<properties/>
</component>
<component id="85201" class="javax.swing.JLabel" binding="testNameLabel">
<constraints>
<grid row="6" column="0" row-span="1" col-span="1" vsize-policy="0" hsize-policy="0" anchor="8" fill="0" indent="0" use-parent-layout="false"/>
</constraints>
<properties>
<labelFor value="d2c23"/>
<text value="Test &name:"/>
</properties>
</component>
<component id="d2c23" class="javax.swing.JTextField" binding="testName">
<constraints>
<grid row="6" column="1" row-span="1" col-span="1" vsize-policy="0" hsize-policy="6" anchor="8" fill="1" indent="0" use-parent-layout="false">
<preferred-size width="150" height="-1"/>
</grid>
</constraints>
<properties/>
</component>
<component id="1da83" class="javax.swing.JLabel">
<constraints>
<grid row="1" column="1" row-span="1" col-span="1" vsize-policy="0" hsize-policy="0" anchor="8" fill="0" indent="1" use-parent-layout="false"/>
</constraints>
<properties>
<enabled value="false"/>
<text value="Scope of the test: either file, directory, or tests in a file and filtered by name."/>
</properties>
</component>
<component id="2ce2f" class="javax.swing.JLabel" binding="testFileHintLabel">
<constraints>
<grid row="3" column="1" row-span="1" col-span="1" vsize-policy="0" hsize-policy="0" anchor="8" fill="0" indent="0" use-parent-layout="false"/>
</constraints>
<properties>
<enabled value="false"/>
<text value="The entry-point for the test (e.g. 'test/simple_test.dart')."/>
</properties>
</component>
<component id="9ab03" class="javax.swing.JLabel" binding="testDirHintLabel">
<constraints>
<grid row="5" column="1" row-span="1" col-span="1" vsize-policy="0" hsize-policy="0" anchor="8" fill="0" indent="0" use-parent-layout="false"/>
</constraints>
<properties>
<enabled value="false"/>
<text value="The directory scoping the run tests (e.g. '/.../test/')."/>
</properties>
</component>
<component id="965ee" class="javax.swing.JLabel" binding="testNameHintLabel">
<constraints>
<grid row="7" column="1" row-span="1" col-span="1" vsize-policy="0" hsize-policy="0" anchor="8" fill="0" indent="0" use-parent-layout="false"/>
</constraints>
<properties>
<enabled value="false"/>
<text value="Some pattern to match test names by."/>
</properties>
</component>
<component id="c35e" class="javax.swing.JLabel">
<constraints>
<grid row="8" column="0" row-span="1" col-span="1" vsize-policy="0" hsize-policy="0" anchor="8" fill="0" indent="0" use-parent-layout="false"/>
</constraints>
<properties>
<labelFor value="88888"/>
<text value="Additional args:"/>
</properties>
</component>
<component id="88888" class="com.intellij.ui.components.fields.ExpandableTextField" binding="additionalArgs">
<constraints>
<grid row="8" column="1" row-span="1" col-span="1" vsize-policy="0" hsize-policy="6" anchor="8" fill="1" indent="0" use-parent-layout="false">
<preferred-size width="150" height="-1"/>
</grid>
</constraints>
<properties/>
</component>
<component id="ff408" class="javax.swing.JLabel">
<constraints>
<grid row="9" column="1" row-span="1" col-span="1" vsize-policy="0" hsize-policy="0" anchor="8" fill="0" indent="0" use-parent-layout="false"/>
</constraints>
<properties>
<enabled value="false"/>
<text value="Additional arguments to pass to the test runner."/>
</properties>
</component>
</children>
</grid>
</form>
| flutter-intellij/flutter-idea/src/io/flutter/run/test/TestForm.form/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/run/test/TestForm.form",
"repo_id": "flutter-intellij",
"token_count": 3162
} | 456 |
/*
* Copyright 2016 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.sdk;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonPrimitive;
import com.google.gson.JsonSyntaxException;
import com.intellij.execution.process.*;
import com.intellij.openapi.diagnostic.Logger;
import com.intellij.openapi.fileEditor.FileDocumentManager;
import com.intellij.openapi.module.Module;
import com.intellij.openapi.project.Project;
import com.intellij.openapi.roots.OrderRootType;
import com.intellij.openapi.roots.libraries.Library;
import com.intellij.openapi.roots.libraries.LibraryTable;
import com.intellij.openapi.roots.libraries.LibraryTablesRegistrar;
import com.intellij.openapi.util.Key;
import com.intellij.openapi.util.io.FileUtil;
import com.intellij.openapi.util.text.StringUtil;
import com.intellij.openapi.vcs.VcsException;
import com.intellij.openapi.vfs.LocalFileSystem;
import com.intellij.openapi.vfs.VfsUtil;
import com.intellij.openapi.vfs.VirtualFile;
import com.intellij.openapi.vfs.VirtualFileManager;
import com.intellij.util.ui.EdtInvocationManager;
import com.jetbrains.lang.dart.sdk.DartSdk;
import git4idea.config.GitExecutableManager;
import io.flutter.FlutterBundle;
import io.flutter.FlutterUtils;
import io.flutter.dart.DartPlugin;
import io.flutter.module.FlutterProjectType;
import io.flutter.pub.PubRoot;
import io.flutter.run.FlutterDevice;
import io.flutter.run.FlutterLaunchMode;
import io.flutter.run.common.RunMode;
import io.flutter.run.test.TestFields;
import io.flutter.settings.FlutterSettings;
import io.flutter.utils.JsonUtils;
import org.jetbrains.annotations.NonNls;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.nio.file.attribute.FileTime;
import java.util.*;
import static java.util.Arrays.asList;
public class FlutterSdk {
public static final String FLUTTER_SDK_GLOBAL_LIB_NAME = "Flutter SDK";
public static final String DART_SDK_SUFFIX = "/bin/cache/dart-sdk";
public static final String LINUX_DART_SUFFIX = "/google-dartlang";
public static final String LOCAL_DART_SUFFIX = "/google-dartlang-local";
public static final String MAC_DART_SUFFIX = "/dart_lang/macos_sdk";
private static final String DART_CORE_SUFFIX = DART_SDK_SUFFIX + "/lib/core";
private static final Logger LOG = Logger.getInstance(FlutterSdk.class);
private static final Map<String, FlutterSdk> projectSdkCache = new HashMap<>();
private final @NotNull VirtualFile myHome;
private final @NotNull FlutterSdkVersion myVersion;
private final Map<String, String> cachedConfigValues = new HashMap<>();
private FlutterSdk(@NotNull final VirtualFile home, @NotNull final FlutterSdkVersion version) {
myHome = home;
myVersion = version;
}
public boolean isOlderThanToolsStamp(@NotNull VirtualFile gen) {
final VirtualFile bin = myHome.findChild("bin");
if (bin == null) return false;
final VirtualFile cache = bin.findChild("cache");
if (cache == null) return false;
final VirtualFile stamp = cache.findChild("flutter_tools.stamp");
if (stamp == null) return false;
try {
final FileTime genFile = Files.getLastModifiedTime(Paths.get(gen.getPath()));
final FileTime stampFile = Files.getLastModifiedTime(Paths.get(stamp.getPath()));
return genFile.compareTo(stampFile) > 0;
}
catch (IOException ignored) {
return false;
}
}
/**
* Return the FlutterSdk for the given project.
* <p>
* Returns null if the Dart SDK is not set or does not exist.
*/
@Nullable
public static FlutterSdk getFlutterSdk(@NotNull final Project project) {
if (project.isDisposed()) {
return null;
}
final DartSdk dartSdk = DartPlugin.getDartSdk(project);
if (dartSdk == null) {
return null;
}
final String dartPath = dartSdk.getHomePath();
if (!dartPath.endsWith(DART_SDK_SUFFIX)) {
return null;
}
final String sdkPath = dartPath.substring(0, dartPath.length() - DART_SDK_SUFFIX.length());
return FlutterSdk.forPath(sdkPath);
}
/**
* Returns the Flutter SDK for a project that has a possibly broken "Dart SDK" project library.
* <p>
* (This can happen for a newly-cloned Flutter SDK where the Dart SDK is not cached yet.)
*/
@Nullable
public static FlutterSdk getIncomplete(@NotNull final Project project) {
if (project.isDisposed()) {
return null;
}
final Library lib = getDartSdkLibrary(project);
if (lib == null) {
return null;
}
return getFlutterFromDartSdkLibrary(lib);
}
@Nullable
public static FlutterSdk forPath(@NotNull final String path) {
final VirtualFile home = LocalFileSystem.getInstance().findFileByPath(path);
if (home == null || !FlutterSdkUtil.isFlutterSdkHome(path)) {
return null;
}
else {
return saveSdkInCache(home);
}
}
@NotNull
private static FlutterSdk saveSdkInCache(VirtualFile home) {
final String cacheKey = home.getCanonicalPath();
synchronized (projectSdkCache) {
if (!projectSdkCache.containsKey(cacheKey)) {
projectSdkCache.put(cacheKey, new FlutterSdk(home, FlutterSdkVersion.readFromSdk(home)));
}
}
return projectSdkCache.get(cacheKey);
}
@Nullable
private static Library getDartSdkLibrary(@NotNull Project project) {
final LibraryTable libraryTable = LibraryTablesRegistrar.getInstance().getLibraryTable(project);
for (Library lib : libraryTable.getLibraries()) {
if ("Dart SDK".equals(lib.getName())) {
return lib;
}
}
return null;
}
@Nullable
private static FlutterSdk getFlutterFromDartSdkLibrary(Library lib) {
final String[] urls = lib.getUrls(OrderRootType.CLASSES);
for (String url : urls) {
if (url.endsWith(DART_CORE_SUFFIX)) {
final String flutterUrl = url.substring(0, url.length() - DART_CORE_SUFFIX.length());
final VirtualFile home = VirtualFileManager.getInstance().findFileByUrl(flutterUrl);
return home == null ? null : saveSdkInCache(home);
}
}
return null;
}
public FlutterCommand flutterVersion() {
// TODO(devoncarew): Switch to calling 'flutter --version --machine'. The ouput will look like:
// Building flutter tool...
// {
// "frameworkVersion": "1.15.4-pre.249",
// "channel": "master",
// "repositoryUrl": "https://github.com/flutter/flutter",
// "frameworkRevision": "3551a51df48743ebd4faa91cc5e3d23db645bdce",
// "frameworkCommitDate": "2020-03-03 08:19:06 +0800",
// "engineRevision": "5e474ee860a3dfa5970a6c54b1cb584152f9c86f",
// "dartSdkVersion": "2.8.0 (build 2.8.0-dev.10.0 fbe9f6115d)"
// }
return new FlutterCommand(this, getHome(), FlutterCommand.Type.VERSION);
}
public FlutterCommand flutterUpgrade() {
return new FlutterCommand(this, getHome(), FlutterCommand.Type.UPGRADE);
}
public FlutterCommand flutterClean(@NotNull PubRoot root) {
return new FlutterCommand(this, root.getRoot(), FlutterCommand.Type.CLEAN);
}
public FlutterCommand flutterDoctor() {
return new FlutterCommand(this, getHome(), FlutterCommand.Type.DOCTOR);
}
@NonNls
public FlutterCommand flutterCreate(@NotNull VirtualFile appDir, @Nullable FlutterCreateAdditionalSettings additionalSettings) {
final List<String> args = new ArrayList<>();
if (additionalSettings != null) {
args.addAll(additionalSettings.getArgs());
if (FlutterProjectType.PLUGIN.equals(additionalSettings.getType()) && getVersion().flutterCreateSupportsPlatforms()) {
// TODO(messick): Remove this after the wizard UI is updated.
if (!args.contains("--platforms")) {
args.add("--platforms");
args.add("android,ios");
}
}
if (getVersion().stableChannelSupportsPlatforms()) {
// The --project-name arg was actually introduced before --platforms but everyone should be on 2.0 by now anyway.
final String projectName = additionalSettings.getProjectName();
if (projectName != null) {
args.add("--project-name");
args.add(projectName);
}
}
}
// keep as the last argument
args.add(appDir.getName());
final String[] vargs = args.toArray(new String[0]);
return new FlutterCommand(this, appDir.getParent(), FlutterCommand.Type.CREATE, vargs);
}
public FlutterCommand flutterPackagesGet(@NotNull PubRoot root) {
return new FlutterCommand(this, root.getRoot(), FlutterCommand.Type.PUB_GET);
}
public FlutterCommand flutterPackagesUpgrade(@NotNull PubRoot root) {
return new FlutterCommand(this, root.getRoot(), FlutterCommand.Type.PUB_UPGRADE);
}
public FlutterCommand flutterPackagesOutdated(@NotNull PubRoot root) {
return new FlutterCommand(this, root.getRoot(), FlutterCommand.Type.PUB_OUTDATED);
}
public FlutterCommand flutterPub(@Nullable PubRoot root, String... args) {
return new FlutterCommand(this, root == null ? null : root.getRoot(), FlutterCommand.Type.PUB, args);
}
public FlutterCommand flutterBuild(@NotNull PubRoot root, String... additionalArgs) {
return new FlutterCommand(this, root.getRoot(), FlutterCommand.Type.BUILD, additionalArgs);
}
public FlutterCommand flutterConfig(String... additionalArgs) {
return new FlutterCommand(this, getHome(), FlutterCommand.Type.CONFIG, additionalArgs);
}
public FlutterCommand flutterChannel() {
return new FlutterCommand(this, getHome(), FlutterCommand.Type.CHANNEL);
}
public FlutterCommand flutterRun(@NotNull PubRoot root,
@NotNull VirtualFile main,
@NotNull FlutterDevice device,
@NotNull RunMode mode,
@NotNull FlutterLaunchMode flutterLaunchMode,
@NotNull Project project,
String... additionalArgs) {
final List<String> args = new ArrayList<>();
args.add("--machine");
if (FlutterSettings.getInstance().isVerboseLogging()) {
args.add("--verbose");
}
if (flutterLaunchMode == FlutterLaunchMode.DEBUG) {
if (getVersion().isTrackWidgetCreationRecommended()) {
// Ensure additionalArgs doesn't have any arg like 'track-widget-creation'.
if (Arrays.stream(additionalArgs).noneMatch(s -> s.contains("track-widget-creation"))) {
args.add("--track-widget-creation");
}
}
}
args.add("--device-id=" + device.deviceId());
// TODO (helin24): Remove special handling for web-server if we can fix https://github.com/flutter/flutter-intellij/issues/4767.
// Currently we can't connect to the VM service for the web-server 'device' to resume.
if (mode == RunMode.DEBUG || (mode == RunMode.RUN && !device.deviceId().equals("web-server"))) {
args.add("--start-paused");
}
if (flutterLaunchMode == FlutterLaunchMode.PROFILE) {
args.add("--profile");
}
else if (flutterLaunchMode == FlutterLaunchMode.RELEASE) {
args.add("--release");
}
args.addAll(asList(additionalArgs));
// Make the path to main relative (to make the command line prettier).
final String mainPath = root.getRelativePath(main);
if (mainPath == null) {
throw new IllegalArgumentException("main isn't within the pub root: " + main.getPath());
}
args.add(FileUtil.toSystemDependentName(mainPath));
return new FlutterCommand(this, root.getRoot(), FlutterCommand.Type.RUN, args.toArray(new String[]{ }));
}
public FlutterCommand flutterAttach(@NotNull PubRoot root, @NotNull VirtualFile main, @Nullable FlutterDevice device,
@NotNull FlutterLaunchMode flutterLaunchMode, String... additionalArgs) {
final List<String> args = new ArrayList<>();
args.add("--machine");
if (FlutterSettings.getInstance().isVerboseLogging()) {
args.add("--verbose");
}
// TODO(messick): Check that 'flutter attach' supports these arguments.
if (flutterLaunchMode == FlutterLaunchMode.PROFILE) {
args.add("--profile");
}
else if (flutterLaunchMode == FlutterLaunchMode.RELEASE) {
args.add("--release");
}
if (device != null) {
args.add("--device-id=" + device.deviceId());
}
// TODO(messick): Add others (target, debug-port).
args.addAll(asList(additionalArgs));
// Make the path to main relative (to make the command line prettier).
final String mainPath = root.getRelativePath(main);
if (mainPath == null) {
throw new IllegalArgumentException("main isn't within the pub root: " + main.getPath());
}
args.add(FileUtil.toSystemDependentName(mainPath));
return new FlutterCommand(this, root.getRoot(), FlutterCommand.Type.ATTACH, args.toArray(new String[]{ }));
}
public FlutterCommand flutterRunOnTester(@NotNull PubRoot root, @NotNull String mainPath) {
final List<String> args = new ArrayList<>();
args.add("--machine");
args.add("--device-id=flutter-tester");
args.add(mainPath);
return new FlutterCommand(this, root.getRoot(), FlutterCommand.Type.RUN, args.toArray(new String[]{ }));
}
public FlutterCommand flutterTest(@NotNull PubRoot root, @NotNull VirtualFile fileOrDir, @Nullable String testNameSubstring,
@NotNull RunMode mode, @Nullable String additionalArgs, TestFields.Scope scope, boolean useRegexp) {
final List<String> args = new ArrayList<>();
if (myVersion.flutterTestSupportsMachineMode()) {
args.add("--machine");
// Otherwise, just run it normally and show the output in a non-test console.
}
if (mode == RunMode.DEBUG) {
if (!myVersion.flutterTestSupportsMachineMode()) {
throw new IllegalStateException("Flutter SDK is too old to debug tests");
}
}
// Starting the app paused so the IDE can catch early errors is ideal. However, we don't have a way to resume for multiple test files
// yet, so we want to exclude directory scope tests from starting paused. See https://github.com/flutter/flutter-intellij/issues/4737.
if (mode == RunMode.DEBUG || (mode == RunMode.RUN && !scope.equals(TestFields.Scope.DIRECTORY))) {
args.add("--start-paused");
}
if (FlutterSettings.getInstance().isVerboseLogging()) {
args.add("--verbose");
}
if (testNameSubstring != null) {
if (!myVersion.flutterTestSupportsFiltering()) {
throw new IllegalStateException("Flutter SDK is too old to select tests by name");
}
if (useRegexp) {
args.add("--name");
args.add(StringUtil.escapeToRegexp(testNameSubstring) + "(\\s*\\(variant: .*\\))?$");
}
else {
args.add("--plain-name");
args.add(testNameSubstring);
}
}
if (additionalArgs != null && !additionalArgs.trim().isEmpty()) {
args.addAll(Arrays.asList(additionalArgs.trim().split(" ")));
}
if (mode == RunMode.COVERAGE) {
if (!args.contains("--coverage")) {
args.add("--coverage");
}
}
if (!root.getRoot().equals(fileOrDir)) {
// Make the path to main relative (to make the command line prettier).
final String mainPath = root.getRelativePath(fileOrDir);
if (mainPath == null) {
throw new IllegalArgumentException("main isn't within the pub root: " + fileOrDir.getPath());
}
args.add(FileUtil.toSystemDependentName(mainPath));
}
return new FlutterCommand(this, root.getRoot(), FlutterCommand.Type.TEST, args.toArray(new String[]{ }));
}
/**
* Runs flutter create and waits for it to finish.
* <p>
* Shows output in a console unless the module parameter is null.
* <p>
* Notifies process listener if one is specified.
* <p>
* Returns the PubRoot if successful.
*/
@Nullable
public PubRoot createFiles(@NotNull VirtualFile baseDir, @Nullable Module module, @Nullable ProcessListener listener,
@Nullable FlutterCreateAdditionalSettings additionalSettings) {
final Process process;
if (module == null) {
process = flutterCreate(baseDir, additionalSettings).start(null, listener);
}
else {
process = flutterCreate(baseDir, additionalSettings).startInModuleConsole(module, null, listener);
}
if (process == null) {
return null;
}
try {
if (process.waitFor() != 0) {
return null;
}
}
catch (InterruptedException e) {
FlutterUtils.warn(LOG, e);
return null;
}
if (EdtInvocationManager.getInstance().isEventDispatchThread()) {
VfsUtil.markDirtyAndRefresh(false, true, true, baseDir); // Need this for AS.
}
else {
baseDir.refresh(false, true); // The current thread must NOT be in a read action.
}
return PubRoot.forDirectory(baseDir);
}
/**
* Starts running 'flutter pub get' on the given pub root provided it's in one of this project's modules.
* <p>
* Shows output in the console associated with the given module.
* <p>
* Returns the process if successfully started.
*/
public Process startPubGet(@NotNull PubRoot root, @NotNull Project project) {
final Module module = root.getModule(project);
if (module == null) return null;
// Ensure pubspec is saved.
FileDocumentManager.getInstance().saveAllDocuments();
// Refresh afterwards to ensure Dart Plugin doesn't mistakenly nag to run pub.
return flutterPackagesGet(root).startInModuleConsole(module, root::refresh, null);
}
/**
* Starts running 'flutter pub upgrade' on the given pub root.
* <p>
* Shows output in the console associated with the given module.
* <p>
* Returns the process if successfully started.
*/
public Process startPubUpgrade(@NotNull PubRoot root, @NotNull Project project) {
final Module module = root.getModule(project);
if (module == null) return null;
// Ensure pubspec is saved.
FileDocumentManager.getInstance().saveAllDocuments();
return flutterPackagesUpgrade(root).startInModuleConsole(module, root::refresh, null);
}
/**
* Starts running 'flutter pub outdated' on the given pub root.
* <p>
* Shows output in the console associated with the given module.
* <p>
* Returns the process if successfully started.
*/
public Process startPubOutdated(@NotNull PubRoot root, @NotNull Project project) {
final Module module = root.getModule(project);
if (module == null) return null;
// Ensure pubspec is saved.
FileDocumentManager.getInstance().saveAllDocuments();
return flutterPackagesOutdated(root).startInModuleConsole(module, root::refresh, null);
}
@NotNull
public VirtualFile getHome() {
return myHome;
}
@NotNull
public String getHomePath() {
return myHome.getPath();
}
/**
* Returns the Flutter Version as captured in the 'version' file. This version is very coarse grained and not meant for presentation and
* rather only for sanity-checking the presence of baseline features (e.g, hot-reload).
*/
@NotNull
public FlutterSdkVersion getVersion() {
return myVersion;
}
/**
* Returns the path to the Dart SDK cached within the Flutter SDK, or null if it doesn't exist.
*/
@Nullable
public String getDartSdkPath() {
return FlutterSdkUtil.pathToDartSdk(getHomePath());
}
@Nullable
@NonNls
public FlutterSdkChannel queryFlutterChannel(boolean useCachedValue) {
if (useCachedValue) {
final String channel = cachedConfigValues.get("channel");
if (channel != null) {
return FlutterSdkChannel.fromText(channel);
}
}
final VirtualFile dir = LocalFileSystem.getInstance().findFileByPath(getHomePath());
assert dir != null;
String branch;
try {
branch = git4idea.light.LightGitUtilKt.getLocation(dir, GitExecutableManager.getInstance().getExecutable((Project)null));
}
catch (VcsException e) {
final String stdout = returnOutputOfQuery(flutterChannel());
if (stdout == null) {
branch = "unknown";
}
else {
branch = FlutterSdkChannel.parseChannel(stdout);
}
}
cachedConfigValues.put("channel", branch);
return FlutterSdkChannel.fromText(branch);
}
@NonNls
private static final String[] PLATFORMS =
new String[]{"enable-android", "enable-ios", "enable-web", "enable-linux-desktop", "enable-macos-desktop", "enable-windows-desktop"};
@NotNull
@NonNls
public Set<String> queryConfiguredPlatforms(boolean useCachedValue) {
final Set<String> platforms = new HashSet<>();
// Someone could do: flutter config --no-enable-ios --no-enable-android
platforms.add("enable-android");
platforms.add("enable-ios");
if (useCachedValue) {
for (String key : PLATFORMS) {
final String value = cachedConfigValues.get(key);
if ("true".equals(value)) {
platforms.add(key);
}
else if ("false".equals(value)) {
platforms.remove(key);
}
}
return platforms;
}
final String stdout = returnOutputOfQuery(flutterConfig("--machine"));
if (stdout == null) {
return platforms;
}
final int startJsonIndex = stdout.indexOf('{');
if (startJsonIndex == -1) {
return platforms;
}
try {
final JsonElement elem = JsonUtils.parseString(stdout.substring(startJsonIndex));
if (elem.isJsonNull()) {
FlutterUtils.warn(LOG, FlutterBundle.message("flutter.sdk.invalid.json.error"));
return platforms;
}
final JsonObject obj = elem.getAsJsonObject();
for (String key : PLATFORMS) {
final JsonPrimitive primitive = obj.getAsJsonPrimitive(key);
if (primitive != null) {
if ("true".equals(primitive.getAsString())) {
platforms.add(key);
}
else if ("false".equals(primitive.getAsString())) {
platforms.remove(key);
}
cachedConfigValues.put(key, primitive.getAsString());
}
}
}
catch (JsonSyntaxException ignored) {
}
return platforms;
}
/**
* Query 'flutter config' for the given key, and optionally use any existing cached value.
*/
@Nullable
public String queryFlutterConfig(String key, boolean useCachedValue) {
if (useCachedValue && cachedConfigValues.containsKey(key)) {
return cachedConfigValues.get(key);
}
final String stdout = returnOutputOfQuery(flutterConfig("--machine"));
if (stdout != null) {
try {
final JsonElement elem = JsonUtils.parseString(stdout.substring(stdout.indexOf('{')));
if (elem.isJsonNull()) {
FlutterUtils.warn(LOG, FlutterBundle.message("flutter.sdk.invalid.json.error"));
return null;
}
final JsonObject obj = elem.getAsJsonObject();
for (String jsonKey : JsonUtils.getKeySet(obj)) {
final JsonElement element = obj.get(jsonKey);
if (element == null || element.isJsonNull()) {
continue;
}
final JsonPrimitive primitive = (JsonPrimitive)element;
cachedConfigValues.put(jsonKey, primitive.getAsString());
}
}
catch (JsonSyntaxException ignored) {
}
}
return null;
}
// Do not run this on EDT.
@Nullable
private String returnOutputOfQuery(@NotNull FlutterCommand command) {
final ColoredProcessHandler process = command.startProcess(false);
if (process == null) {
return null;
}
final StringBuilder stdout = new StringBuilder();
process.addProcessListener(new ProcessAdapter() {
@Override
public void onTextAvailable(@NotNull ProcessEvent event, @NotNull Key outputType) {
if (outputType == ProcessOutputTypes.STDOUT) {
stdout.append(event.getText());
}
}
});
LOG.info("Calling " + command.getDisplayCommand());
final long start = System.currentTimeMillis();
process.startNotify();
if (process.waitFor(5000)) {
final long duration = System.currentTimeMillis() - start;
LOG.info(command.getDisplayCommand() + ": " + duration + "ms");
final Integer code = process.getExitCode();
if (code != null && code == 0) {
return stdout.toString();
}
else {
LOG.info("Exit code from " + command.getDisplayCommand() + ": " + code);
}
}
else {
LOG.info("Timeout when calling " + command.getDisplayCommand());
}
return null;
}
}
| flutter-intellij/flutter-idea/src/io/flutter/sdk/FlutterSdk.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/sdk/FlutterSdk.java",
"repo_id": "flutter-intellij",
"token_count": 9219
} | 457 |
package io.flutter.test;
import com.google.gson.*;
import com.intellij.execution.testframework.TestConsoleProperties;
import com.intellij.execution.testframework.sm.ServiceMessageBuilder;
import com.intellij.execution.testframework.sm.runner.OutputToGeneralTestEventsConverter;
import com.intellij.openapi.diagnostic.Logger;
import com.intellij.openapi.util.Key;
import com.intellij.openapi.util.text.StringUtil;
import com.intellij.openapi.vfs.VirtualFile;
import com.intellij.util.PathUtil;
import com.jetbrains.lang.dart.ide.runner.util.DartTestLocationProvider;
import com.jetbrains.lang.dart.util.DartUrlResolver;
import gnu.trove.TIntLongHashMap;
import io.flutter.utils.JsonUtils;
import jetbrains.buildServer.messages.serviceMessages.ServiceMessageVisitor;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.lang.reflect.Type;
import java.text.ParseException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* Convert events from JSON format generated by package:test to the string format
* expected by the event processor.
* NOTE: The test runner runs tests asynchronously. It is possible to get a 'testDone'
* event followed some time later by an 'error' event for that same test. That should
* convert a successful test into a failure. That case is not being handled.
*/
@SuppressWarnings({"Duplicates", "FieldMayBeFinal", "LocalCanBeFinal", "SameReturnValue"})
public class DartTestEventsConverterZ extends OutputToGeneralTestEventsConverter {
private static final Logger LOG = Logger.getInstance(DartTestEventsConverterZ.class);
private static final String TYPE_START = "start";
private static final String TYPE_SUITE = "suite";
private static final String TYPE_ERROR = "error";
private static final String TYPE_GROUP = "group";
private static final String TYPE_PRINT = "print";
private static final String TYPE_DONE = "done";
private static final String TYPE_ALL_SUITES = "allSuites";
private static final String TYPE_TEST_START = "testStart";
private static final String TYPE_TEST_DONE = "testDone";
private static final String DEF_GROUP = "group";
private static final String DEF_SUITE = "suite";
private static final String DEF_TEST = "test";
private static final String DEF_METADATA = "metadata";
private static final String JSON_TYPE = "type";
private static final String JSON_NAME = "name";
private static final String JSON_ID = "id";
private static final String JSON_TEST_ID = "testID";
private static final String JSON_SUITE_ID = "suiteID";
private static final String JSON_PARENT_ID = "parentID";
private static final String JSON_GROUP_IDS = "groupIDs";
private static final String JSON_RESULT = "result";
private static final String JSON_MILLIS = "time";
private static final String JSON_COUNT = "count";
private static final String JSON_TEST_COUNT = "testCount";
private static final String JSON_MESSAGE = "message";
private static final String JSON_ERROR_MESSAGE = "error";
private static final String JSON_STACK_TRACE = "stackTrace";
private static final String JSON_PATH = "path";
private static final String JSON_PLATFORM = "platform";
private static final String JSON_LINE = "line";
private static final String JSON_COLUMN = "column";
private static final String JSON_URL = "url";
private static final String JSON_ROOT_LINE = "root_line";
private static final String JSON_ROOT_COLUMN = "root_column";
private static final String JSON_ROOT_URL = "root_url";
private static final String RESULT_SUCCESS = "success";
private static final String RESULT_FAILURE = "failure";
private static final String RESULT_ERROR = "error";
private static final String EXPECTED = "Expected: ";
private static final Pattern EXPECTED_ACTUAL_RESULT = Pattern.compile("\\nExpected: (.*)\\n Actual: (.*)\\n *\\^\\n Differ.*\\n");
private static final String FILE_URL_PREFIX = "dart_location://";
private static final String LOADING_PREFIX = "loading ";
private static final String COMPILING_PREFIX = "compiling ";
private static final String SET_UP_ALL_VIRTUAL_TEST_NAME = "(setUpAll)";
private static final String TEAR_DOWN_ALL_VIRTUAL_TEST_NAME = "(tearDownAll)";
private static final Gson GSON = new Gson();
@NotNull private final DartUrlResolver myUrlResolver;
private String myLocation;
private Key myCurrentOutputType;
private ServiceMessageVisitor myCurrentVisitor;
private final TIntLongHashMap myTestIdToTimestamp;
private final Map<Integer, Test> myTestData;
private final Map<Integer, Group> myGroupData;
private final Map<Integer, Suite> mySuiteData;
private int mySuitCount;
public DartTestEventsConverterZ(@NotNull final String testFrameworkName,
@NotNull final TestConsoleProperties consoleProperties,
@NotNull final DartUrlResolver urlResolver) {
super(testFrameworkName, consoleProperties);
myUrlResolver = urlResolver;
myTestIdToTimestamp = new TIntLongHashMap();
myTestData = new HashMap<>();
myGroupData = new HashMap<>();
mySuiteData = new HashMap<>();
}
@Override
protected boolean processServiceMessages(final String text, final @NotNull Key outputType, final @NotNull ServiceMessageVisitor visitor)
throws ParseException {
LOG.debug("<<< " + text.trim());
myCurrentOutputType = outputType;
myCurrentVisitor = visitor;
// service message parser expects line like "##teamcity[ .... ]" without whitespaces in the end.
return processEventText(text);
}
@SuppressWarnings("SimplifiableIfStatement")
private boolean processEventText(final String text) throws JsonSyntaxException, ParseException {
JsonElement elem;
try {
elem = JsonUtils.parseString(text);
}
catch (JsonSyntaxException ex) {
if (text.contains("\"json\" is not an allowed value for option \"reporter\"")) {
final ServiceMessageBuilder testStarted = ServiceMessageBuilder.testStarted("Failed to start");
final ServiceMessageBuilder testFailed = ServiceMessageBuilder.testFailed("Failed to start");
testFailed.addAttribute("message", "Please update your pubspec.yaml dependency on package:test to version 0.12.9 or later.");
final ServiceMessageBuilder testFinished = ServiceMessageBuilder.testFinished("Failed to start");
return finishMessage(testStarted, 1, 0) & finishMessage(testFailed, 1, 0) & finishMessage(testFinished, 1, 0);
}
return doProcessServiceMessages(text);
}
if (elem != null && elem.isJsonArray()) return process(elem.getAsJsonArray());
if (elem == null || !elem.isJsonObject()) return false;
return process(elem.getAsJsonObject());
}
/**
* Hook to process arrays.
*/
protected boolean process(JsonArray array) {
return false;
}
private boolean doProcessServiceMessages(@NotNull final String text) throws ParseException {
LOG.debug(">>> " + text);
return super.processServiceMessages(text, myCurrentOutputType, myCurrentVisitor);
}
@SuppressWarnings("SimplifiableIfStatement")
private boolean process(JsonObject obj) throws JsonSyntaxException, ParseException {
String type = obj.get(JSON_TYPE).getAsString();
if (TYPE_TEST_START.equals(type)) {
return handleTestStart(obj);
}
else if (TYPE_TEST_DONE.equals(type)) {
return handleTestDone(obj);
}
else if (TYPE_ERROR.equals(type)) {
return handleError(obj);
}
else if (TYPE_PRINT.equals(type)) {
return handlePrint(obj);
}
else if (TYPE_GROUP.equals(type)) {
return handleGroup(obj);
}
else if (TYPE_SUITE.equals(type)) {
return handleSuite(obj);
}
else if (TYPE_ALL_SUITES.equals(type)) {
return handleAllSuites(obj);
}
else if (TYPE_START.equals(type)) {
return handleStart(obj);
}
else if (TYPE_DONE.equals(type)) {
return handleDone(obj);
}
else {
return true;
}
}
private boolean handleTestStart(JsonObject obj) throws ParseException {
final JsonObject testObj = obj.getAsJsonObject(DEF_TEST);
// Not reached if testObj == null.
final Test test = getTest(obj);
myTestIdToTimestamp.put(test.getId(), getTimestamp(obj));
if (shouldTestBeHiddenIfPassed(test)) {
// Virtual test that represents loading or compiling a test suite. See lib/src/runner/loader.dart -> Loader.loadFile() in pkg/test source code
// At this point we do not report anything to the framework, but if error occurs, we'll report it as a normal test
String path = "";
if (test.getName().startsWith(LOADING_PREFIX)) {
path = test.getName().substring(LOADING_PREFIX.length());
}
else if (test.getName().startsWith(COMPILING_PREFIX)) {
path = test.getName().substring(COMPILING_PREFIX.length());
}
if (!path.isEmpty()) myLocation = FILE_URL_PREFIX + path;
test.myTestStartReported = false;
return true;
}
final ServiceMessageBuilder testStarted = ServiceMessageBuilder.testStarted(test.getBaseName());
test.myTestStartReported = true;
preprocessTestStart(test);
addLocationHint(testStarted, test);
boolean result = finishMessage(testStarted, test.getId(), test.getValidParentId());
final Metadata metadata = Metadata.from(testObj.getAsJsonObject(DEF_METADATA));
if (metadata.skip) {
final ServiceMessageBuilder message = ServiceMessageBuilder.testIgnored(test.getBaseName());
if (metadata.skipReason != null) message.addAttribute("message", metadata.skipReason);
result &= finishMessage(message, test.getId(), test.getValidParentId());
}
return result;
}
/**
* Hook to preprocess tests before adding location info and generating a service message.
*/
protected void preprocessTestStart(@NotNull Test test) {
}
private static boolean shouldTestBeHiddenIfPassed(@NotNull final Test test) {
// There are so called 'virtual' tests that are created for loading test suites, setUpAll(), and tearDownAll().
// They shouldn't be visible when they do not cause problems. But if any error occurs, we'll report it later as a normal test.
// See lib/src/runner/loader.dart -> Loader.loadFile() and lib/src/backend/declarer.dart -> Declarer._setUpAll and Declarer._tearDownAll in pkg/test source code
final Group group = test.getParent();
return group == null && (test.getName().startsWith(LOADING_PREFIX) || test.getName().startsWith(COMPILING_PREFIX))
||
group != null && group.getDoneTestsCount() == 0 && test.getBaseName().equals(SET_UP_ALL_VIRTUAL_TEST_NAME)
||
group != null && group.getDoneTestsCount() > 0 && test.getBaseName().equals(TEAR_DOWN_ALL_VIRTUAL_TEST_NAME);
}
private boolean handleTestDone(JsonObject obj) throws ParseException {
final Test test = getTest(obj);
if (!test.myTestStartReported) return true;
String result = getResult(obj);
if (!result.equals(RESULT_SUCCESS) && !result.equals(RESULT_FAILURE) && !result.equals(RESULT_ERROR)) {
throw new ParseException("Unknown result: " + obj, 0);
}
test.testDone();
//if (test.getMetadata().skip) return true; // skipped tests are reported as ignored in handleTestStart(). testFinished signal must follow
ServiceMessageBuilder testFinished = ServiceMessageBuilder.testFinished(test.getBaseName());
long duration = getTimestamp(obj) - myTestIdToTimestamp.get(test.getId());
testFinished.addAttribute("duration", Long.toString(duration));
return finishMessage(testFinished, test.getId(), test.getValidParentId()) && checkGroupDone(test.getParent());
}
@SuppressWarnings("SimplifiableIfStatement")
private boolean checkGroupDone(@Nullable final Group group) throws ParseException {
if (group != null && group.getTestCount() > 0 && group.getDoneTestsCount() == group.getTestCount()) {
return processGroupDone(group) && checkGroupDone(group.getParent());
}
return true;
}
private boolean handleGroup(JsonObject obj) throws ParseException {
final Group group = getGroup(obj.getAsJsonObject(DEF_GROUP));
return handleGroup(group);
}
protected boolean handleGroup(@NotNull Group group) throws ParseException {
// From spec: The implicit group at the root of each test suite has null name and parentID attributes.
if (group.getParent() == null && group.getTestCount() > 0) {
// com.intellij.execution.testframework.sm.runner.OutputToGeneralTestEventsConverter.MyServiceMessageVisitor.KEY_TESTS_COUNT
// and com.intellij.execution.testframework.sm.runner.OutputToGeneralTestEventsConverter.MyServiceMessageVisitor.ATTR_KEY_TEST_COUNT
final ServiceMessageBuilder testCount =
new ServiceMessageBuilder("testCount").addAttribute("count", String.valueOf(group.getTestCount()));
doProcessServiceMessages(testCount.toString());
}
if (group.isArtificial()) return true; // Ignore artificial groups.
ServiceMessageBuilder groupMsg = ServiceMessageBuilder.testSuiteStarted(group.getBaseName());
// Possible attributes: "nodeType" "nodeArgs" "running"
addLocationHint(groupMsg, group);
return finishMessage(groupMsg, group.getId(), group.getValidParentId());
}
private boolean handleSuite(JsonObject obj) throws ParseException {
Suite suite = getSuite(obj.getAsJsonObject(DEF_SUITE));
if (!suite.hasPath()) {
mySuiteData.remove(suite.getId());
}
return true;
}
private boolean handleError(JsonObject obj) throws ParseException {
final Test test = getTest(obj);
final String message = getErrorMessage(obj);
boolean result = true;
if (!test.myTestStartReported) {
final ServiceMessageBuilder testStarted = ServiceMessageBuilder.testStarted(test.getBaseName());
test.myTestStartReported = true;
result = finishMessage(testStarted, test.getId(), test.getValidParentId());
}
if (test.myTestErrorReported) {
final ServiceMessageBuilder testErrorMessage = ServiceMessageBuilder.testStdErr(test.getBaseName());
testErrorMessage.addAttribute("out", appendLineBreakIfNeeded(message));
result &= finishMessage(testErrorMessage, test.getId(), test.getValidParentId());
}
else {
final ServiceMessageBuilder testError = ServiceMessageBuilder.testFailed(test.getBaseName());
test.myTestErrorReported = true;
String failureMessage = message;
int firstExpectedIndex = message.indexOf(EXPECTED);
if (firstExpectedIndex >= 0) {
Matcher matcher = EXPECTED_ACTUAL_RESULT.matcher(message);
if (matcher.find(firstExpectedIndex + EXPECTED.length())) {
String expectedText = matcher.group(1);
String actualText = matcher.group(2);
testError.addAttribute("expected", expectedText);
testError.addAttribute("actual", actualText);
if (firstExpectedIndex == 0) {
failureMessage = "Comparison failed";
}
else {
failureMessage = message.substring(0, firstExpectedIndex);
}
}
}
testError.addAttribute("message", appendLineBreakIfNeeded(failureMessage));
result &= finishMessage(testError, test.getId(), test.getValidParentId());
}
final String stackTrace = getStackTrace(obj);
if (!StringUtil.isEmptyOrSpaces(stackTrace)) {
final ServiceMessageBuilder stackTraceMessage = ServiceMessageBuilder.testStdErr(test.getBaseName());
stackTraceMessage.addAttribute("out", appendLineBreakIfNeeded(stackTrace));
result &= finishMessage(stackTraceMessage, test.getId(), test.getValidParentId());
}
return result;
}
@NotNull
private static String appendLineBreakIfNeeded(@NotNull final String message) {
return message.endsWith("\n") ? message : message + "\n";
}
private boolean handleAllSuites(JsonObject obj) {
JsonElement elem = obj.get(JSON_COUNT);
if (elem == null || !elem.isJsonPrimitive()) return true;
mySuitCount = elem.getAsInt();
return true;
}
private boolean handlePrint(JsonObject obj) throws ParseException {
final Test test = getTest(obj);
boolean result = true;
if (!test.myTestStartReported) {
if (test.getBaseName().equals(SET_UP_ALL_VIRTUAL_TEST_NAME) || test.getBaseName().equals(TEAR_DOWN_ALL_VIRTUAL_TEST_NAME)) {
return true; // output in successfully passing setUpAll/tearDownAll is not important enough to make these nodes visible
}
final ServiceMessageBuilder testStarted = ServiceMessageBuilder.testStarted(test.getBaseName());
test.myTestStartReported = true;
result = finishMessage(testStarted, test.getId(), test.getValidParentId());
}
ServiceMessageBuilder message = ServiceMessageBuilder.testStdOut(test.getBaseName());
message.addAttribute("out", appendLineBreakIfNeeded(getMessage(obj)));
return result & finishMessage(message, test.getId(), test.getValidParentId());
}
private boolean handleStart(JsonObject obj) throws ParseException {
myTestIdToTimestamp.clear();
myTestData.clear();
myGroupData.clear();
mySuiteData.clear();
mySuitCount = 0;
// TODO: Change to ServiceMessageBuilder.testsStarted() for 2020.1.
return doProcessServiceMessages(new ServiceMessageBuilder("enteredTheMatrix").toString());
}
@SuppressWarnings("RedundantThrows")
private boolean handleDone(JsonObject obj) throws ParseException {
// The test runner has reached the end of the tests.
processAllTestsDone();
return true;
}
private void processAllTestsDone() {
// All tests are done.
for (Group group : myGroupData.values()) {
// For package: test prior to v. 0.12.9 there were no Group.testCount field, so need to finish them all at the end.
// AFAIK the order does not matter. A depth-first post-order traversal of the tree would work
// if order does matter. Note: Currently, there is no tree representation, just parent links.
if (group.getTestCount() == 0 || group.getDoneTestsCount() != group.getTestCount()) {
try {
processGroupDone(group);
}
catch (ParseException ex) {
// ignore it
}
}
}
myTestIdToTimestamp.clear();
myTestData.clear();
myGroupData.clear();
mySuiteData.clear();
mySuitCount = 0;
}
private boolean processGroupDone(@NotNull final Group group) throws ParseException {
if (group.isArtificial()) return true;
ServiceMessageBuilder groupMsg = ServiceMessageBuilder.testSuiteFinished(group.getBaseName());
return finishMessage(groupMsg, group.getId(), group.getValidParentId());
}
private boolean finishMessage(@NotNull ServiceMessageBuilder msg, int testId, int parentId) throws ParseException {
msg.addAttribute("nodeId", String.valueOf(testId));
msg.addAttribute("parentNodeId", String.valueOf(parentId));
return doProcessServiceMessages(msg.toString());
}
private void addLocationHint(ServiceMessageBuilder messageBuilder, Item item) {
String location = "unknown";
String loc;
final boolean badUrl = item.getUrl() == null || item.getUrl().endsWith(".dart.js");
final VirtualFile file = badUrl ? null : myUrlResolver.findFileByDartUrl(item.getUrl());
if (file != null) {
loc = FILE_URL_PREFIX + file.getPath();
}
else if (item.hasSuite()) {
loc = FILE_URL_PREFIX + item.getSuite().getPath();
}
else {
loc = myLocation;
}
if (loc != null) {
if (badUrl) {
loc += ",-1,-1";
}
else {
loc += "," + item.getLine() + "," + item.getColumn();
}
String nameList = GSON.toJson(item.nameList(), DartTestLocationProvider.STRING_LIST_TYPE);
location = loc + "," + nameList;
}
messageBuilder.addAttribute("locationHint", location);
}
private static long getTimestamp(JsonObject obj) throws ParseException {
return getLong(obj, JSON_MILLIS);
}
private static long getLong(JsonObject obj, String name) throws ParseException {
JsonElement val = obj == null ? null : obj.get(name);
if (val == null || !val.isJsonPrimitive()) throw new ParseException("Value is not type long: " + val, 0);
return val.getAsLong();
}
private static boolean getBoolean(JsonObject obj, String name) throws ParseException {
JsonElement val = obj == null ? null : obj.get(name);
if (val == null || !val.isJsonPrimitive()) throw new ParseException("Value is not type boolean: " + val, 0);
return val.getAsBoolean();
}
@NotNull
private Test getTest(JsonObject obj) throws ParseException {
return getItem(obj, myTestData);
}
@NotNull
private Group getGroup(JsonObject obj) throws ParseException {
return getItem(obj, myGroupData);
}
@NotNull
private Suite getSuite(JsonObject obj) throws ParseException {
return getItem(obj, mySuiteData);
}
@NotNull
private <T extends Item> T getItem(JsonObject obj, Map<Integer, T> items) throws ParseException {
if (obj == null) throw new ParseException("Unexpected null json object", 0);
T item;
JsonElement id = obj.get(JSON_ID);
if (id != null) {
if (items == myTestData) {
@SuppressWarnings("unchecked") T type = (T)Test.from(obj, myGroupData, mySuiteData);
item = type;
}
else if (items == myGroupData) {
@SuppressWarnings("unchecked") T group = (T)Group.from(obj, myGroupData, mySuiteData);
item = group;
}
else {
@SuppressWarnings("unchecked") T suite = (T)Suite.from(obj);
item = suite;
}
items.put(id.getAsInt(), item);
}
else {
JsonElement testId = obj.get(JSON_TEST_ID);
if (testId != null) {
int baseId = testId.getAsInt();
item = items.get(baseId);
}
else {
JsonElement testObj = obj.get(DEF_TEST);
if (testObj != null) {
return getItem(testObj.getAsJsonObject(), items);
}
else {
throw new ParseException("No testId in json object", 0);
}
}
}
return item;
}
@NotNull
private static String getErrorMessage(JsonObject obj) {
return nonNullJsonValue(obj, JSON_ERROR_MESSAGE, "<no error message>");
}
@NotNull
private static String getMessage(JsonObject obj) {
return nonNullJsonValue(obj, JSON_MESSAGE, "<no message>");
}
@NotNull
private static String getStackTrace(JsonObject obj) {
return nonNullJsonValue(obj, JSON_STACK_TRACE, "<no stack trace>");
}
@NotNull
private static String getResult(JsonObject obj) {
return nonNullJsonValue(obj, JSON_RESULT, "<no result>");
}
@NotNull
private static String nonNullJsonValue(JsonObject obj, @NotNull String id, @NotNull String def) {
JsonElement val = obj == null ? null : obj.get(id);
if (val == null || !val.isJsonPrimitive()) return def;
return val.getAsString();
}
protected static class Item {
protected static final String NO_NAME = "<no name>";
private final int myId;
// Visible and mutable to allow for processing.
public String myName;
public Group myParent;
public String myUrl;
private final Suite mySuite;
private final Metadata myMetadata;
private final int myLine;
private final int myColumn;
static int extractInt(JsonObject obj, String memberName) {
JsonElement elem = obj.get(memberName);
if (elem == null || !elem.isJsonPrimitive()) return -1;
return elem.getAsInt();
}
static String extractString(JsonObject obj, String memberName, String defaultResult) {
JsonElement elem = obj.get(memberName);
if (elem == null || elem.isJsonNull()) return defaultResult;
return elem.getAsString();
}
static Metadata extractMetadata(JsonObject obj) {
return Metadata.from(obj.get(DEF_METADATA));
}
static Suite lookupSuite(JsonObject obj, Map<Integer, Suite> suites) {
JsonElement suiteObj = obj.get(JSON_SUITE_ID);
Suite suite = null;
if (suiteObj != null && suiteObj.isJsonPrimitive()) {
int parentId = suiteObj.getAsInt();
suite = suites.get(parentId);
}
return suite;
}
Item(int id, String name, Group parent, Suite suite, Metadata metadata, int line, int column, String url) {
myId = id;
myName = name;
myParent = parent;
mySuite = suite;
myMetadata = metadata;
myLine = line;
myColumn = column;
myUrl = url;
}
int getId() {
return myId;
}
public String getName() {
return myName;
}
String getBaseName() {
// Virtual test that represents loading or compiling a test suite. See lib/src/runner/loader.dart -> Loader.loadFile() in pkg/test source code
if (this instanceof Test && getParent() == null) {
if (myName.startsWith(LOADING_PREFIX)) {
return LOADING_PREFIX + PathUtil.getFileName(myName.substring(LOADING_PREFIX.length()));
}
else if (myName.startsWith(COMPILING_PREFIX)) {
return COMPILING_PREFIX + PathUtil.getFileName(myName.substring(COMPILING_PREFIX.length()));
}
return myName; // can't happen
}
// file-level group
if (this instanceof Group && NO_NAME.equals(myName) && myParent == null && hasSuite()) {
return PathUtil.getFileName(getSuite().getPath());
}
// top-level group in suite
if (this instanceof Group && myParent != null && myParent.getParent() == null && NO_NAME.equals(myParent.getName())) {
return myName;
}
if (hasValidParent()) {
final String parentName = getParent().getName();
if (myName.startsWith(parentName + " ")) {
return myName.substring(parentName.length() + 1);
}
}
return myName;
}
boolean hasSuite() {
return mySuite != null && mySuite.hasPath();
}
public Suite getSuite() {
return mySuite;
}
Group getParent() {
return myParent;
}
Metadata getMetadata() {
return myMetadata;
}
boolean isArtificial() {
return NO_NAME.equals(myName) && myParent == null && !hasSuite();
}
boolean hasValidParent() {
return !(myParent == null || myParent.isArtificial());
}
int getValidParentId() {
if (hasValidParent()) {
return getParent().getId();
}
else {
return 0;
}
}
List<String> nameList() {
List<String> names = new ArrayList<>();
addNames(names);
return names;
}
void addNames(List<String> names) {
if (this instanceof Group && NO_NAME.equals(myName) && myParent == null) {
return; // do not add a name of a file-level group
}
if (myParent != null) {
myParent.addNames(names);
}
names.add(StringUtil.escapeStringCharacters(getBaseName()));
}
public int getLine() {
return myLine;
}
public int getColumn() {
return myColumn;
}
public String getUrl() {
return myUrl;
}
public String toString() {
return getClass().getSimpleName() + "(" + myId + "," + myName + ")";
}
}
protected static class Test extends Item {
private boolean myTestStartReported = false;
private boolean myTestErrorReported = false;
static Test from(JsonObject obj, Map<Integer, Group> groups, Map<Integer, Suite> suites) {
int[] groupIds = GSON.fromJson(obj.get(JSON_GROUP_IDS), (Type)int[].class);
Group parent = null;
if (groupIds != null && groupIds.length > 0) {
parent = groups.get(groupIds[groupIds.length - 1]);
}
Suite suite = lookupSuite(obj, suites);
int line = extractInt(obj, JSON_ROOT_LINE);
if (line < 0) line = extractInt(obj, JSON_LINE);
int column = extractInt(obj, JSON_ROOT_COLUMN);
if (column < 0) column = extractInt(obj, JSON_COLUMN);
String url = extractString(obj, JSON_ROOT_URL, null);
if (url == null) url = extractString(obj, JSON_URL, null);
return new Test(extractInt(obj, JSON_ID), extractString(obj, JSON_NAME, NO_NAME), parent, suite, extractMetadata(obj),
line < 0 ? -1 : line - 1, column < 0 ? -1 : column - 1, url);
}
Test(int id, String name, Group parent, Suite suite, Metadata metadata, int line, int column, String url) {
super(id, name, parent, suite, metadata, line, column, url);
}
public void testDone() {
if (getParent() != null) {
getParent().incDoneTestsCount();
}
}
}
protected static class Group extends Item {
private int myTestCount;
private int myDoneTestsCount = 0;
static Group from(JsonObject obj, Map<Integer, Group> groups, Map<Integer, Suite> suites) {
JsonElement parentObj = obj.get(JSON_PARENT_ID);
Group parent = null;
if (parentObj != null && parentObj.isJsonPrimitive()) {
int parentId = parentObj.getAsInt();
parent = groups.get(parentId);
}
Suite suite = lookupSuite(obj, suites);
final int line = extractInt(obj, JSON_LINE);
final int column = extractInt(obj, JSON_COLUMN);
String groupName = extractString(obj, JSON_NAME, "");
if (groupName.isEmpty()) {
groupName = NO_NAME;
}
return new Group(extractInt(obj, JSON_ID), groupName, parent, suite, extractMetadata(obj),
extractInt(obj, JSON_TEST_COUNT), line < 0 ? -1 : line - 1, column < 0 ? -1 : column - 1,
extractString(obj, JSON_URL, null));
}
Group(int id, String name, Group parent, Suite suite, Metadata metadata, int count, int line, int column, String url) {
super(id, name, parent, suite, metadata, line, column, url);
myTestCount = count;
}
int getTestCount() {
return myTestCount;
}
public int getDoneTestsCount() {
return myDoneTestsCount;
}
public void incDoneTestsCount() {
myDoneTestsCount++;
if (getParent() != null) {
getParent().incDoneTestsCount();
}
}
}
protected static class Suite extends Item {
static Metadata NoMetadata = new Metadata();
static String NONE = "<none>";
static Suite from(JsonObject obj) {
return new Suite(extractInt(obj, JSON_ID), extractString(obj, JSON_PATH, NONE), extractString(obj, JSON_PLATFORM, NONE));
}
private final String myPlatform;
Suite(int id, String path, String platform) {
super(id, path, null, null, NoMetadata, -1, -1, "file://" + path);
myPlatform = platform;
}
String getPath() {
return getName();
}
String getPlatform() {
return myPlatform;
}
@SuppressWarnings("StringEquality")
boolean hasPath() {
return getPath() != NONE;
}
}
private static class Metadata {
@SuppressWarnings("unused") private boolean skip; // assigned by GSON via reflection
@SuppressWarnings("unused") private String skipReason; // assigned by GSON via reflection
static Metadata from(JsonElement elem) {
if (elem == null) return new Metadata();
return GSON.fromJson(elem, (Type)Metadata.class);
}
}
}
| flutter-intellij/flutter-idea/src/io/flutter/test/DartTestEventsConverterZ.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/test/DartTestEventsConverterZ.java",
"repo_id": "flutter-intellij",
"token_count": 11264
} | 458 |
/*
* Copyright 2021 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.utils;
import com.intellij.lang.ASTNode;
import com.intellij.openapi.diagnostic.Logger;
import com.intellij.openapi.vfs.LocalFileSystem;
import com.intellij.openapi.vfs.VirtualFile;
import com.intellij.psi.PsiElement;
import com.intellij.psi.impl.source.tree.AstBufferUtil;
import com.intellij.util.TripleFunction;
import com.jetbrains.lang.dart.DartTokenTypes;
import com.jetbrains.lang.dart.psi.DartComponent;
import com.jetbrains.lang.dart.psi.DartFile;
import io.flutter.FlutterUtils;
import java.awt.AlphaComposite;
import java.awt.Color;
import java.awt.Font;
import java.awt.FontFormatException;
import java.awt.Graphics2D;
import java.awt.RenderingHints;
import java.awt.font.FontRenderContext;
import java.awt.font.LineMetrics;
import java.awt.geom.AffineTransform;
import java.awt.geom.Rectangle2D;
import java.awt.image.BufferedImage;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.URL;
import java.nio.file.Paths;
import java.util.Properties;
import javax.imageio.ImageIO;
import javax.swing.Icon;
import javax.swing.ImageIcon;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
@SuppressWarnings("UseJBColor")
public class IconPreviewGenerator {
private static final Logger LOG = Logger.getInstance(IconPreviewGenerator.class);
@NotNull final String fontFilePath;
int iconSize = 16;
int fontSize = 16;
@NotNull Color fontColor = Color.gray;
public IconPreviewGenerator(@NotNull String fontFilePath) {
this.fontFilePath = fontFilePath;
}
public IconPreviewGenerator(@NotNull String fontFilePath, int iconSize, int fontSize, @Nullable Color fontColor) {
this(fontFilePath);
this.iconSize = iconSize;
this.fontSize = fontSize;
if (fontColor != null) {
this.fontColor = fontColor;
}
}
public Icon convert(String number) {
int codepoint;
if (number.startsWith("0x") || number.startsWith("0X")) {
number = number.substring(2);
codepoint = Integer.parseInt(number, 16);
}
else {
codepoint = Integer.parseInt(number, 10);
}
return convert(codepoint);
}
public Icon convert(int code) {
return runInGraphicsContext((BufferedImage image, Graphics2D graphics, FontRenderContext frc) -> {
char ch = Character.toChars(code)[0];
String codepoint = Character.toString(ch);
drawGlyph(codepoint, graphics, frc);
return new ImageIcon(image);
});
}
// Given a file at path-to-font-properties in the format generated by tools_metadata (on github),
// to generate double-size icons for all glyphs in a font:
// IconPreviewGenerator ipg = new IconPreviewGenerator("path-to-ttf-file", 32, 32, Color.black);
// ipg.batchConvert("path-to-out-dir", "path-to-font-properties", "@2x");
public void batchConvert(@NotNull String outPath, @NotNull String path, @NotNull String suffix) {
final String outputPath = outPath.endsWith("/") ? outPath : outPath + "/";
//noinspection ResultOfMethodCallIgnored
new File(outputPath).mkdirs();
runInGraphicsContext((BufferedImage image, Graphics2D graphics, FontRenderContext frc) -> {
Properties fontMap = new Properties();
File file = new File(path);
try (InputStream stream = new BufferedInputStream(new FileInputStream(file))) {
fontMap.load(stream);
for (Object nextKey : fontMap.keySet()) {
if (!(nextKey instanceof String)) continue;
String codepoint = (String)nextKey;
if (!codepoint.endsWith(".codepoint")) continue;
String iconName = fontMap.getProperty(codepoint);
codepoint = codepoint.substring(0, codepoint.indexOf(".codepoint"));
int code = Integer.parseInt(codepoint, 16);
char ch = Character.toChars(code)[0];
codepoint = Character.toString(ch);
drawGlyph(codepoint, graphics, frc);
ImageIO.write(image, "PNG", new File(outputPath + iconName + suffix + ".png"));
}
}
catch (IOException ex) {
FlutterUtils.warn(LOG, ex);
}
return null;
});
}
private Icon runInGraphicsContext(TripleFunction<BufferedImage, Graphics2D, FontRenderContext, Icon> callback) {
Icon result = null;
Graphics2D graphics = null;
//noinspection UndesirableClassUsage
BufferedImage image = new BufferedImage(iconSize, iconSize, BufferedImage.TYPE_4BYTE_ABGR);
try (InputStream inputStream = new FileInputStream(fontFilePath)) {
Font font = Font.createFont(Font.TRUETYPE_FONT, inputStream).deriveFont(Font.PLAIN, fontSize);
graphics = image.createGraphics();
graphics.setFont(font);
graphics.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
FontRenderContext frc = new FontRenderContext(new AffineTransform(), true, true);
result = callback.fun(image, graphics, frc);
}
catch (IOException | FontFormatException ex) {
FlutterUtils.warn(LOG, ex);
}
finally {
if (graphics != null) graphics.dispose();
}
return result;
}
private void drawGlyph(String codepoint, Graphics2D graphics, FontRenderContext frc) {
Font font = graphics.getFont();
Rectangle2D rect = font.getStringBounds(codepoint, frc);
LineMetrics metrics = font.getLineMetrics(codepoint, frc);
float lineHeight = metrics.getHeight();
float ascent = metrics.getAscent();
float width = (float)rect.getWidth();
float x0 = (iconSize - width) / 2.0f;
float y0 = (iconSize - lineHeight) / 2.0f + ascent + (lineHeight - ascent) / 2.0f;
graphics.setComposite(AlphaComposite.Clear);
graphics.fillRect(0, 0, iconSize, iconSize);
graphics.setComposite(AlphaComposite.Src);
graphics.setColor(fontColor);
graphics.drawString(codepoint, x0, y0);
}
@Nullable
public static VirtualFile findAssetMapFor(@NotNull DartComponent dartClass) {
final ASTNode node = dartClass.getNode();
if (DartTokenTypes.CLASS_DEFINITION != node.getElementType()) {
return null;
}
final DartFile psiElement = (DartFile)node.getPsi().getParent();
final VirtualFile file = psiElement.getVirtualFile();
VirtualFile map = findAssetMapIn(file.getParent());
if (map != null) {
return map;
}
final PsiElement identifier = dartClass.getNameIdentifier();
if (identifier == null) {
return null;
}
final String className = AstBufferUtil.getTextSkippingWhitespaceComments(identifier.getNode());
final URL resource = IconPreviewGenerator.class.getResource("/iconAssetMaps/" + className + "/asset_map.yaml");
if (resource == null) {
return null;
}
try {
final URI uri = resource.toURI();
return LocalFileSystem.getInstance().findFileByNioFile(Paths.get(uri));
}
catch (URISyntaxException e) {
return null;
}
}
@Nullable
public static VirtualFile findAssetMapIn(@NotNull VirtualFile dartClass) {
VirtualFile dir = dartClass.getParent();
VirtualFile preview = null;
while (dir != null && !dir.getName().equals("lib")) {
preview = dir.findChild("ide_preview");
if (preview == null) {
dir = dir.getParent();
}
else {
dir = null;
}
}
if (preview == null && dir != null && dir.getName().equals("lib")) {
dir = dir.getParent();
preview = dir.findChild("ide_preview");
if (preview == null) {
return null;
}
}
return preview == null ? null : preview.findChild("asset_map.yaml");
}
}
| flutter-intellij/flutter-idea/src/io/flutter/utils/IconPreviewGenerator.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/utils/IconPreviewGenerator.java",
"repo_id": "flutter-intellij",
"token_count": 2909
} | 459 |
/*
* Copyright 2018 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.utils.animation;
/**
* A mapping of the unit interval to the unit interval.
* <p>
* A curve must map t=0.0 to 0.0 and t=1.0 to 1.0.
*/
public abstract class Curve {
/// Returns the value of the curve at point `t`.
///
/// The value of `t` must be between 0.0 and 1.0, inclusive. Subclasses should
/// assert that this is true.
///
/// A curve must map t=0.0 to 0.0 and t=1.0 to 1.0.
public abstract double transform(double t);
public double interpolate(double start, double end, double t) {
final double fraction = transform(t);
return start * (1 - fraction) + end * fraction;
}
public int interpolate(int start, int end, double t) {
return (int)Math.round(interpolate((double)start, (double)end, t));
}
/// Returns a new curve that is the reversed inversion of this one.
/// This is often useful as the reverseCurve of an [Animation].
///
/// 
/// 
///
/// See also:
///
/// * [FlippedCurve], the class that is used to implement this getter.
public Curve getFlipped() {
return new FlippedCurve(this);
}
@Override
public String toString() {
return getClass().toString();
}
} | flutter-intellij/flutter-idea/src/io/flutter/utils/animation/Curve.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/utils/animation/Curve.java",
"repo_id": "flutter-intellij",
"token_count": 492
} | 460 |
/*
* Copyright 2023 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.view;
import com.intellij.ui.content.ContentManager;
import javax.swing.*;
public interface EmbeddedTab {
void loadUrl(String url);
void close();
JComponent getTabComponent(ContentManager contentManager);
}
| flutter-intellij/flutter-idea/src/io/flutter/view/EmbeddedTab.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/view/EmbeddedTab.java",
"repo_id": "flutter-intellij",
"token_count": 118
} | 461 |
/*
* Copyright 2018 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.view;
import com.intellij.icons.AllIcons;
import io.flutter.run.daemon.FlutterApp;
import io.flutter.vmService.ServiceExtensions;
import org.jetbrains.annotations.NotNull;
public class PerformanceOverlayAction extends FlutterViewToggleableAction<Boolean> {
public PerformanceOverlayAction(@NotNull FlutterApp app) {
super(app, AllIcons.Nodes.PpLib, ServiceExtensions.performanceOverlay);
}
}
| flutter-intellij/flutter-idea/src/io/flutter/view/PerformanceOverlayAction.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/view/PerformanceOverlayAction.java",
"repo_id": "flutter-intellij",
"token_count": 183
} | 462 |
/*
* Copyright 2019 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.vmService;
import java.util.Arrays;
import java.util.List;
public class ToggleableServiceExtensionDescription<T> extends ServiceExtensionDescription<T> {
public ToggleableServiceExtensionDescription(
String extension,
String description,
T enabledValue,
T disabledValue,
String enabledText,
String disabledText
) {
super(extension,
description,
Arrays.asList(enabledValue, disabledValue),
Arrays.asList(enabledText, disabledText));
}
static int enabledIndex = 0;
static int disabledIndex = 1;
public T getEnabledValue() {
final List<T> values = super.getValues();
return values.get(enabledIndex);
}
public T getDisabledValue() {
final List<T> values = super.getValues();
return values.get(disabledIndex);
}
public String getEnabledText() {
final List<String> tooltips = super.getTooltips();
return tooltips.get(enabledIndex);
}
public String getDisabledText() {
final List<String> tooltips = super.getTooltips();
return tooltips.get(disabledIndex);
}
}
| flutter-intellij/flutter-idea/src/io/flutter/vmService/ToggleableServiceExtensionDescription.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/io/flutter/vmService/ToggleableServiceExtensionDescription.java",
"repo_id": "flutter-intellij",
"token_count": 410
} | 463 |
/*
* Copyright (c) 2019, the Dart project authors. Please see the AUTHORS file
* for details. All rights reserved. Use of this source code is governed by a
* BSD-style license that can be found in the LICENSE file.
*
* This file has been automatically generated. Please do not edit it manually.
* To regenerate the file, use the script "pkg/analysis_server/tool/spec/generate_files".
*/
package org.dartlang.analysis.server.protocol;
/**
* An enumeration of the kinds of FlutterOutline elements. The list of kinds might be expanded with
* time, clients must be able to handle new kinds in some general way.
*
* @coverage dart.server.generated.types
*/
public class FlutterOutlineKind {
/**
* A dart element declaration.
*/
public static final String DART_ELEMENT = "DART_ELEMENT";
/**
* A generic Flutter element, without additional information.
*/
public static final String GENERIC = "GENERIC";
/**
* A new instance creation.
*/
public static final String NEW_INSTANCE = "NEW_INSTANCE";
/**
* An invocation of a method, a top-level function, a function expression, etc.
*/
public static final String INVOCATION = "INVOCATION";
/**
* A reference to a local variable, or a field.
*/
public static final String VARIABLE = "VARIABLE";
/**
* The parent node has a required Widget. The node works as a placeholder child to drop a new
* Widget to.
*/
public static final String PLACEHOLDER = "PLACEHOLDER";
}
| flutter-intellij/flutter-idea/src/org/dartlang/analysis/server/protocol/FlutterOutlineKind.java/0 | {
"file_path": "flutter-intellij/flutter-idea/src/org/dartlang/analysis/server/protocol/FlutterOutlineKind.java",
"repo_id": "flutter-intellij",
"token_count": 435
} | 464 |
/*
* Copyright 2020 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.analytics;
import org.jetbrains.annotations.NotNull;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
public class MockAnalyticsTransport implements Analytics.Transport {
final public List<Map<String, String>> sentValues = new ArrayList<>();
@Override
public void send(@NotNull String url, @NotNull Map<String, String> values) {
sentValues.add(values);
}
}
| flutter-intellij/flutter-idea/testSrc/unit/io/flutter/analytics/MockAnalyticsTransport.java/0 | {
"file_path": "flutter-intellij/flutter-idea/testSrc/unit/io/flutter/analytics/MockAnalyticsTransport.java",
"repo_id": "flutter-intellij",
"token_count": 176
} | 465 |
/*
* Copyright 2021 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.ide;
import com.intellij.openapi.application.WriteAction;
import com.intellij.openapi.module.Module;
import com.intellij.openapi.project.Project;
import com.intellij.openapi.roots.ModuleRootManager;
import com.intellij.openapi.roots.ModuleRootModificationUtil;
import com.intellij.openapi.vfs.VirtualFile;
import com.intellij.psi.PsiElement;
import com.intellij.testFramework.fixtures.BasePlatformTestCase;
import com.intellij.testFramework.fixtures.IdeaProjectTestFixture;
import org.jetbrains.annotations.NotNull;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
/**
* Inspired by similar class in the Dart plugin.
*/
@SuppressWarnings("ConstantConditions")
abstract public class FlutterCodeInsightFixtureTestCase extends BasePlatformTestCase implements IdeaProjectTestFixture {
@Override
public void setUp() throws Exception {
super.setUp();
WriteAction.runAndWait(() -> {
try {
final ModuleRootManager rootManager = ModuleRootManager.getInstance(getModule());
if (rootManager != null) {
final VirtualFile[] allContentRoots = rootManager.getContentRoots();
final VirtualFile contentRoot = allContentRoots[0];
final VirtualFile pubspec = contentRoot.createChildData(this, "pubspec.yaml");
pubspec.setBinaryContent(SamplePubspec.getBytes(StandardCharsets.UTF_8));
final VirtualFile dartTool = contentRoot.createChildDirectory(this, ".dart_tool");
final VirtualFile config = dartTool.createChildData(this, "package_config.json");
config.setBinaryContent(SampleConfig.getBytes(StandardCharsets.UTF_8));
}
}
catch (IOException e) {
fail(Arrays.toString(e.getCause().getStackTrace()));
}
});
}
@Override
public void tearDown() throws Exception {
try {
final VirtualFile root = Objects.requireNonNull(ModuleRootManager.getInstance(getModule())).getContentRoots()[0];
assert root != null;
final VirtualFile pubspec = root.findChild("pubspec.yaml");
final VirtualFile dartTool = root.findChild(".dart_tool");
if (pubspec != null && dartTool != null) {
final VirtualFile config = dartTool.findChild("package_config.json");
WriteAction.run(() -> {
pubspec.delete(this);
if (config != null) config.delete(this);
dartTool.delete(this);
});
final List<String> toUnexclude = Arrays.asList(root.getUrl() + "/build", root.getUrl() + "/.pub", root.getUrl() + "/.dart_tool");
ModuleRootModificationUtil.updateExcludedFolders(getModule(), root, toUnexclude, Collections.emptyList());
}
}
catch (Throwable ex) {
addSuppressedException(ex);
}
finally {
super.tearDown();
}
}
/**
* Creates the syntax tree for a Dart file at a specific path and returns the innermost element with the given text.
*/
@NotNull
protected <E extends PsiElement> E setUpDartElement(String filePath, String fileText, String elementText, Class<E> expectedClass) {
assert fileText != null && elementText != null && expectedClass != null && myFixture != null;
return DartTestUtils.setUpDartElement(filePath, fileText, elementText, expectedClass, Objects.requireNonNull(myFixture.getProject()));
}
/**
* Creates the syntax tree for a Dart file and returns the innermost element with the given text.
*/
@NotNull
protected <E extends PsiElement> E setUpDartElement(String fileText, String elementText, Class<E> expectedClass) {
assert fileText != null && elementText != null && expectedClass != null && myFixture != null;
return DartTestUtils.setUpDartElement(null, fileText, elementText, expectedClass, Objects.requireNonNull(myFixture.getProject()));
}
@Override
@NotNull
protected String getTestDataPath() {
return DartTestUtils.BASE_TEST_DATA_PATH + getBasePath();
}
public void addStandardPackage(@NotNull final String packageName) {
assert myFixture != null;
myFixture.copyDirectoryToProject("../packages/" + packageName, "packages/" + packageName);
}
@NotNull
@Override
public Project getProject() {
assert myFixture != null;
return Objects.requireNonNull(myFixture.getProject());
}
@NotNull
@Override
public Module getModule() {
assert myFixture != null;
return Objects.requireNonNull(myFixture.getModule());
}
private static final String SamplePubspec =
"name: hello_world\n" +
"\n" +
"environment:\n" +
" sdk: \">=2.12.0-0 <3.0.0\"\n" +
"\n" +
"dependencies:\n" +
" flutter:\n" +
" sdk: flutter\n";
private static final String SampleConfig =
"{\n" +
" \"configVersion\": 2,\n" +
" \"packages\": [\n" +
" {\n" +
" \"name\": \"cupertino_icons\",\n" +
" \"rootUri\": \"file:///Users/messick/.pub-cache/hosted/pub.dartlang.org/cupertino_icons-1.0.3\",\n" +
" \"packageUri\": \"lib/\",\n" +
" \"languageVersion\": \"2.12\"\n" +
" },\n" +
" {\n" +
" \"name\": \"flutter\",\n" +
" \"rootUri\": \"file:///Users/messick/src/flutter/flutter/packages/flutter\",\n" +
" \"packageUri\": \"lib/\",\n" +
" \"languageVersion\": \"2.12\"\n" +
" },\n" +
" ],\n" +
" \"generated\": \"2021-05-12T16:34:11.007747Z\",\n" +
" \"generator\": \"pub\",\n" +
" \"generatorVersion\": \"2.14.0-48.0.dev\"\n" +
"}";
}
| flutter-intellij/flutter-idea/testSrc/unit/io/flutter/ide/FlutterCodeInsightFixtureTestCase.java/0 | {
"file_path": "flutter-intellij/flutter-idea/testSrc/unit/io/flutter/ide/FlutterCodeInsightFixtureTestCase.java",
"repo_id": "flutter-intellij",
"token_count": 2143
} | 466 |
/*
* Copyright 2019 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.run.bazel;
import io.flutter.testing.ProjectFixture;
import io.flutter.testing.Testing;
import org.junit.Rule;
import org.junit.Test;
import static org.hamcrest.CoreMatchers.equalTo;
import static org.hamcrest.MatcherAssert.assertThat;
/**
* Verify the behavior of bazel run configuration factories.
*
* <p>
* These tests validate preconditions from Bazel IntelliJ plugin logic for how run configurations are saved to Piper.
* If these tests fail, you may need to update some g3 code to prevent breaking g3 Bazel run configurations.
*/
public class BazelConfigurationFactoryTest {
final FlutterBazelRunConfigurationType type = new FlutterBazelRunConfigurationType();
@Rule
public ProjectFixture projectFixture = Testing.makeEmptyModule();
@Test
public void factoryIdIsCorrect() {
// Bazel code assumes the id of the factory as a precondition.
assertThat(type.factory.getId(), equalTo("Flutter (Bazel)"));
}
@Test
public void factoryConfigTypeMatches() {
assertThat(type.getId(), equalTo("FlutterBazelRunConfigurationType"));
}
}
| flutter-intellij/flutter-idea/testSrc/unit/io/flutter/run/bazel/BazelConfigurationFactoryTest.java/0 | {
"file_path": "flutter-intellij/flutter-idea/testSrc/unit/io/flutter/run/bazel/BazelConfigurationFactoryTest.java",
"repo_id": "flutter-intellij",
"token_count": 366
} | 467 |
package io.flutter.utils;
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class UrlUtilsTest {
@Test
public void testGenerateHtmlFragmentWithHrefTags() {
assertEquals(
UrlUtils.generateHtmlFragmentWithHrefTags("Open http://link.com"),
"Open <a href=\"http://link.com\">http://link.com</a>"
);
assertEquals(UrlUtils.generateHtmlFragmentWithHrefTags("Unchanged text without URLs"), "Unchanged text without URLs");
assertEquals(
UrlUtils.generateHtmlFragmentWithHrefTags("Multiple http://link1.com links http://link2.com test"),
"Multiple <a href=\"http://link1.com\">http://link1.com</a> links <a href=\"http://link2.com\">http://link2.com</a> test"
);
}
}
| flutter-intellij/flutter-idea/testSrc/unit/io/flutter/utils/UrlUtilsTest.java/0 | {
"file_path": "flutter-intellij/flutter-idea/testSrc/unit/io/flutter/utils/UrlUtilsTest.java",
"repo_id": "flutter-intellij",
"token_count": 343
} | 468 |
/*
* Copyright (c) 2015, the Dart project authors.
*
* Licensed under the Eclipse Public License v1.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.eclipse.org/legal/epl-v10.html
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package org.dartlang.vm.service.element;
// This file is generated by the script: pkg/vm_service/tool/generate.dart in dart-lang/sdk.
import com.google.gson.JsonArray;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import java.util.List;
/**
* An {@link Event} is an asynchronous notification from the VM. It is delivered only when the
* client has subscribed to an event stream using the streamListen RPC.
*/
@SuppressWarnings({"WeakerAccess", "unused"})
public class Event extends Response {
public Event(JsonObject json) {
super(json);
}
/**
* The alias of the registered service.
*
* This is provided for the event kinds:
* - ServiceRegistered
*
* Can return <code>null</code>.
*/
public String getAlias() {
return getAsString("alias");
}
/**
* Is the isolate paused at an await, yield, or yield* statement?
*
* This is provided for the event kinds:
* - PauseBreakpoint
* - PauseInterrupted
*
* Can return <code>null</code>.
*/
public boolean getAtAsyncSuspension() {
return getAsBoolean("atAsyncSuspension");
}
/**
* The breakpoint which was added, removed, or resolved.
*
* This is provided for the event kinds:
* - PauseBreakpoint
* - BreakpointAdded
* - BreakpointRemoved
* - BreakpointResolved
* - BreakpointUpdated
*
* Can return <code>null</code>.
*/
public Breakpoint getBreakpoint() {
JsonObject obj = (JsonObject) json.get("breakpoint");
if (obj == null) return null;
final String type = json.get("type").getAsString();
if ("Instance".equals(type) || "@Instance".equals(type)) {
final String kind = json.get("kind").getAsString();
if ("Null".equals(kind)) return null;
}
return new Breakpoint(obj);
}
/**
* An array of bytes, encoded as a base64 string.
*
* This is provided for the WriteEvent event.
*
* Can return <code>null</code>.
*/
public String getBytes() {
return getAsString("bytes");
}
/**
* A CPU profile containing recent samples.
*
* Can return <code>null</code>.
*/
public CpuSamplesEvent getCpuSamples() {
JsonObject obj = (JsonObject) json.get("cpuSamples");
if (obj == null) return null;
final String type = json.get("type").getAsString();
if ("Instance".equals(type) || "@Instance".equals(type)) {
final String kind = json.get("kind").getAsString();
if ("Null".equals(kind)) return null;
}
return new CpuSamplesEvent(obj);
}
/**
* The exception associated with this event, if this is a PauseException event.
*
* Can return <code>null</code>.
*/
public InstanceRef getException() {
JsonObject obj = (JsonObject) json.get("exception");
if (obj == null) return null;
return new InstanceRef(obj);
}
/**
* The extension event data.
*
* This is provided for the Extension event.
*
* Can return <code>null</code>.
*/
public ExtensionData getExtensionData() {
JsonObject obj = (JsonObject) json.get("extensionData");
if (obj == null) return null;
final String type = json.get("type").getAsString();
if ("Instance".equals(type) || "@Instance".equals(type)) {
final String kind = json.get("kind").getAsString();
if ("Null".equals(kind)) return null;
}
return new ExtensionData(obj);
}
/**
* The extension event kind.
*
* This is provided for the Extension event.
*
* Can return <code>null</code>.
*/
public String getExtensionKind() {
return getAsString("extensionKind");
}
/**
* The RPC name of the extension that was added.
*
* This is provided for the ServiceExtensionAdded event.
*
* Can return <code>null</code>.
*/
public String getExtensionRPC() {
return getAsString("extensionRPC");
}
/**
* The name of the changed flag.
*
* This is provided for the event kinds:
* - VMFlagUpdate
*
* Can return <code>null</code>.
*/
public String getFlag() {
return getAsString("flag");
}
/**
* The garbage collection (GC) operation performed.
*
* This is provided for the event kinds:
* - GC
*
* Can return <code>null</code>.
*/
public String getGcType() {
return getAsString("gcType");
}
/**
* The argument passed to dart:developer.inspect.
*
* This is provided for the Inspect event.
*
* Can return <code>null</code>.
*/
public InstanceRef getInspectee() {
JsonObject obj = (JsonObject) json.get("inspectee");
if (obj == null) return null;
return new InstanceRef(obj);
}
/**
* The isolate with which this event is associated.
*
* This is provided for all event kinds except for:
* - VMUpdate, VMFlagUpdate
*
* Can return <code>null</code>.
*/
public IsolateRef getIsolate() {
JsonObject obj = (JsonObject) json.get("isolate");
if (obj == null) return null;
final String type = json.get("type").getAsString();
if ("Instance".equals(type) || "@Instance".equals(type)) {
final String kind = json.get("kind").getAsString();
if ("Null".equals(kind)) return null;
}
return new IsolateRef(obj);
}
/**
* What kind of event is this?
*/
public EventKind getKind() {
final JsonElement value = json.get("kind");
try {
return value == null ? EventKind.Unknown : EventKind.valueOf(value.getAsString());
} catch (IllegalArgumentException e) {
return EventKind.Unknown;
}
}
/**
* Specifies whether this event is the last of a group of events.
*
* This is provided for the event kinds:
* - HeapSnapshot
*
* Can return <code>null</code>.
*/
public boolean getLast() {
return getAsBoolean("last");
}
/**
* LogRecord data.
*
* This is provided for the Logging event.
*
* Can return <code>null</code>.
*/
public LogRecord getLogRecord() {
JsonObject obj = (JsonObject) json.get("logRecord");
if (obj == null) return null;
final String type = json.get("type").getAsString();
if ("Instance".equals(type) || "@Instance".equals(type)) {
final String kind = json.get("kind").getAsString();
if ("Null".equals(kind)) return null;
}
return new LogRecord(obj);
}
/**
* The RPC method that should be used to invoke the service.
*
* This is provided for the event kinds:
* - ServiceRegistered
* - ServiceUnregistered
*
* Can return <code>null</code>.
*/
public String getMethod() {
return getAsString("method");
}
/**
* The new value of the changed flag.
*
* This is provided for the event kinds:
* - VMFlagUpdate
*
* Can return <code>null</code>.
*/
public String getNewValue() {
return getAsString("newValue");
}
/**
* The list of breakpoints at which we are currently paused for a PauseBreakpoint event.
*
* This list may be empty. For example, while single-stepping, the VM sends a PauseBreakpoint
* event with no breakpoints.
*
* If there is more than one breakpoint set at the program position, then all of them will be
* provided.
*
* This is provided for the event kinds:
* - PauseBreakpoint
*
* Can return <code>null</code>.
*/
public ElementList<Breakpoint> getPauseBreakpoints() {
if (json.get("pauseBreakpoints") == null) return null;
return new ElementList<Breakpoint>(json.get("pauseBreakpoints").getAsJsonArray()) {
@Override
protected Breakpoint basicGet(JsonArray array, int index) {
return new Breakpoint(array.get(index).getAsJsonObject());
}
};
}
/**
* The previous UserTag label.
*
* Can return <code>null</code>.
*/
public String getPreviousTag() {
return getAsString("previousTag");
}
/**
* The service identifier.
*
* This is provided for the event kinds:
* - ServiceRegistered
* - ServiceUnregistered
*
* Can return <code>null</code>.
*/
public String getService() {
return getAsString("service");
}
/**
* The status (success or failure) related to the event. This is provided for the event kinds:
* - IsolateReloaded
*
* Can return <code>null</code>.
*/
public String getStatus() {
return getAsString("status");
}
/**
* An array of TimelineEvents
*
* This is provided for the TimelineEvents event.
*
* Can return <code>null</code>.
*/
public ElementList<TimelineEvent> getTimelineEvents() {
if (json.get("timelineEvents") == null) return null;
return new ElementList<TimelineEvent>(json.get("timelineEvents").getAsJsonArray()) {
@Override
protected TimelineEvent basicGet(JsonArray array, int index) {
return new TimelineEvent(array.get(index).getAsJsonObject());
}
};
}
/**
* The timestamp (in milliseconds since the epoch) associated with this event. For some isolate
* pause events, the timestamp is from when the isolate was paused. For other events, the
* timestamp is from when the event was created.
*/
public long getTimestamp() {
return json.get("timestamp") == null ? -1 : json.get("timestamp").getAsLong();
}
/**
* The top stack frame associated with this event, if applicable.
*
* This is provided for the event kinds:
* - PauseBreakpoint
* - PauseInterrupted
* - PauseException
*
* For PauseInterrupted events, there will be no top frame if the isolate is idle (waiting in the
* message loop).
*
* For the Resume event, the top frame is provided at all times except for the initial resume
* event that is delivered when an isolate begins execution.
*
* Can return <code>null</code>.
*/
public Frame getTopFrame() {
JsonObject obj = (JsonObject) json.get("topFrame");
if (obj == null) return null;
final String type = json.get("type").getAsString();
if ("Instance".equals(type) || "@Instance".equals(type)) {
final String kind = json.get("kind").getAsString();
if ("Null".equals(kind)) return null;
}
return new Frame(obj);
}
/**
* The new set of recorded timeline streams.
*
* This is provided for the TimelineStreamSubscriptionsUpdate event.
*
* Can return <code>null</code>.
*/
public List<String> getUpdatedStreams() {
return json.get("updatedStreams") == null ? null : getListString("updatedStreams");
}
/**
* The current UserTag label.
*
* Can return <code>null</code>.
*/
public String getUpdatedTag() {
return getAsString("updatedTag");
}
/**
* The vm with which this event is associated.
*
* This is provided for the event kind:
* - VMUpdate, VMFlagUpdate
*
* Can return <code>null</code>.
*/
public VMRef getVm() {
JsonObject obj = (JsonObject) json.get("vm");
if (obj == null) return null;
final String type = json.get("type").getAsString();
if ("Instance".equals(type) || "@Instance".equals(type)) {
final String kind = json.get("kind").getAsString();
if ("Null".equals(kind)) return null;
}
return new VMRef(obj);
}
}
| flutter-intellij/flutter-idea/third_party/vmServiceDrivers/org/dartlang/vm/service/element/Event.java/0 | {
"file_path": "flutter-intellij/flutter-idea/third_party/vmServiceDrivers/org/dartlang/vm/service/element/Event.java",
"repo_id": "flutter-intellij",
"token_count": 4032
} | 469 |
/*
* Copyright (c) 2015, the Dart project authors.
*
* Licensed under the Eclipse Public License v1.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.eclipse.org/legal/epl-v10.html
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package org.dartlang.vm.service.element;
// This file is generated by the script: pkg/vm_service/tool/generate.dart in dart-lang/sdk.
import com.google.gson.JsonArray;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
/**
* {@link InstanceRef} is a reference to an {@link Instance}.
*/
@SuppressWarnings({"WeakerAccess", "unused"})
public class InstanceRef extends ObjRef {
public InstanceRef(JsonObject json) {
super(json);
}
/**
* The stack trace associated with the allocation of a ReceivePort.
*
* Provided for instance kinds:
* - ReceivePort
*
* Can return <code>null</code>.
*/
public InstanceRef getAllocationLocation() {
JsonObject obj = (JsonObject) json.get("allocationLocation");
if (obj == null) return null;
return new InstanceRef(obj);
}
/**
* Instance references always include their class.
*/
public ClassRef getClassRef() {
return new ClassRef((JsonObject) json.get("class"));
}
/**
* The context associated with a Closure instance.
*
* Provided for instance kinds:
* - Closure
*
* Can return <code>null</code>.
*/
public ContextRef getClosureContext() {
JsonObject obj = (JsonObject) json.get("closureContext");
if (obj == null) return null;
final String type = json.get("type").getAsString();
if ("Instance".equals(type) || "@Instance".equals(type)) {
final String kind = json.get("kind").getAsString();
if ("Null".equals(kind)) return null;
}
return new ContextRef(obj);
}
/**
* The function associated with a Closure instance.
*
* Provided for instance kinds:
* - Closure
*
* Can return <code>null</code>.
*/
public FuncRef getClosureFunction() {
JsonObject obj = (JsonObject) json.get("closureFunction");
if (obj == null) return null;
final String type = json.get("type").getAsString();
if ("Instance".equals(type) || "@Instance".equals(type)) {
final String kind = json.get("kind").getAsString();
if ("Null".equals(kind)) return null;
}
return new FuncRef(obj);
}
/**
* A name associated with a ReceivePort used for debugging purposes.
*
* Provided for instance kinds:
* - ReceivePort
*
* Can return <code>null</code>.
*/
public String getDebugName() {
return getAsString("debugName");
}
/**
* The identityHashCode assigned to the allocated object. This hash code is the same as the hash
* code provided in HeapSnapshot and CpuSample's returned by getAllocationTraces().
*/
public int getIdentityHashCode() {
return getAsInt("identityHashCode");
}
/**
* What kind of instance is this?
*/
public InstanceKind getKind() {
final JsonElement value = json.get("kind");
try {
return value == null ? InstanceKind.Unknown : InstanceKind.valueOf(value.getAsString());
} catch (IllegalArgumentException e) {
return InstanceKind.Unknown;
}
}
/**
* The number of (non-static) fields of a PlainInstance, or the length of a List, or the number
* of associations in a Map, or the number of codeunits in a String, or the total number of
* fields (positional and named) in a Record.
*
* Provided for instance kinds:
* - PlainInstance
* - String
* - List
* - Map
* - Set
* - Uint8ClampedList
* - Uint8List
* - Uint16List
* - Uint32List
* - Uint64List
* - Int8List
* - Int16List
* - Int32List
* - Int64List
* - Float32List
* - Float64List
* - Int32x4List
* - Float32x4List
* - Float64x2List
* - Record
*
* Can return <code>null</code>.
*/
public int getLength() {
return getAsInt("length");
}
/**
* The name of a Type instance.
*
* Provided for instance kinds:
* - Type
*
* Can return <code>null</code>.
*/
public String getName() {
return getAsString("name");
}
/**
* The parameterized class of a type parameter.
*
* Provided for instance kinds:
* - TypeParameter
*
* Can return <code>null</code>.
*/
public ClassRef getParameterizedClass() {
JsonObject obj = (JsonObject) json.get("parameterizedClass");
if (obj == null) return null;
final String type = json.get("type").getAsString();
if ("Instance".equals(type) || "@Instance".equals(type)) {
final String kind = json.get("kind").getAsString();
if ("Null".equals(kind)) return null;
}
return new ClassRef(obj);
}
/**
* The list of parameter types for a function.
*
* Provided for instance kinds:
* - FunctionType
*
* Can return <code>null</code>.
*/
public ElementList<Parameter> getParameters() {
if (json.get("parameters") == null) return null;
return new ElementList<Parameter>(json.get("parameters").getAsJsonArray()) {
@Override
protected Parameter basicGet(JsonArray array, int index) {
return new Parameter(array.get(index).getAsJsonObject());
}
};
}
/**
* The pattern of a RegExp instance.
*
* The pattern is always an instance of kind String.
*
* Provided for instance kinds:
* - RegExp
*
* Can return <code>null</code>.
*/
public InstanceRef getPattern() {
JsonObject obj = (JsonObject) json.get("pattern");
if (obj == null) return null;
return new InstanceRef(obj);
}
/**
* The port ID for a ReceivePort.
*
* Provided for instance kinds:
* - ReceivePort
*
* Can return <code>null</code>.
*/
public int getPortId() {
return getAsInt("portId");
}
/**
* The return type of a function.
*
* Provided for instance kinds:
* - FunctionType
*
* Can return <code>null</code>.
*/
public InstanceRef getReturnType() {
JsonObject obj = (JsonObject) json.get("returnType");
if (obj == null) return null;
return new InstanceRef(obj);
}
/**
* The corresponding Class if this Type has a resolved typeClass.
*
* Provided for instance kinds:
* - Type
*
* Can return <code>null</code>.
*/
public ClassRef getTypeClass() {
JsonObject obj = (JsonObject) json.get("typeClass");
if (obj == null) return null;
final String type = json.get("type").getAsString();
if ("Instance".equals(type) || "@Instance".equals(type)) {
final String kind = json.get("kind").getAsString();
if ("Null".equals(kind)) return null;
}
return new ClassRef(obj);
}
/**
* The type parameters for a function.
*
* Provided for instance kinds:
* - FunctionType
*
* Can return <code>null</code>.
*/
public ElementList<InstanceRef> getTypeParameters() {
if (json.get("typeParameters") == null) return null;
return new ElementList<InstanceRef>(json.get("typeParameters").getAsJsonArray()) {
@Override
protected InstanceRef basicGet(JsonArray array, int index) {
return new InstanceRef(array.get(index).getAsJsonObject());
}
};
}
/**
* The value of this instance as a string.
*
* Provided for the instance kinds:
* - Null (null)
* - Bool (true or false)
* - Double (suitable for passing to Double.parse())
* - Int (suitable for passing to int.parse())
* - String (value may be truncated)
* - Float32x4
* - Float64x2
* - Int32x4
* - StackTrace
*
* Can return <code>null</code>.
*/
public String getValueAsString() {
return getAsString("valueAsString");
}
/**
* The valueAsString for String references may be truncated. If so, this property is added with
* the value 'true'.
*
* New code should use 'length' and 'count' instead.
*
* Can return <code>null</code>.
*/
public boolean getValueAsStringIsTruncated() {
final JsonElement elem = json.get("valueAsStringIsTruncated");
return elem != null ? elem.getAsBoolean() : false;
}
/**
* Returns whether this instance represents null.
*/
public boolean isNull() {
return getKind() == InstanceKind.Null;
}
}
| flutter-intellij/flutter-idea/third_party/vmServiceDrivers/org/dartlang/vm/service/element/InstanceRef.java/0 | {
"file_path": "flutter-intellij/flutter-idea/third_party/vmServiceDrivers/org/dartlang/vm/service/element/InstanceRef.java",
"repo_id": "flutter-intellij",
"token_count": 3053
} | 470 |
/*
* Copyright (c) 2015, the Dart project authors.
*
* Licensed under the Eclipse Public License v1.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.eclipse.org/legal/epl-v10.html
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package org.dartlang.vm.service.element;
// This file is generated by the script: pkg/vm_service/tool/generate.dart in dart-lang/sdk.
import com.google.gson.JsonObject;
import java.util.List;
/**
* A {@link Script} provides information about a Dart language script.
*/
@SuppressWarnings({"WeakerAccess", "unused"})
public class Script extends Obj {
public Script(JsonObject json) {
super(json);
}
/**
* Can return <code>null</code>.
*/
public int getColumnOffset() {
return getAsInt("columnOffset");
}
/**
* The library which owns this script.
*/
public LibraryRef getLibrary() {
return new LibraryRef((JsonObject) json.get("library"));
}
/**
* Can return <code>null</code>.
*/
public int getLineOffset() {
return getAsInt("lineOffset");
}
/**
* The source code for this script. This can be null for certain built-in scripts.
*
* Can return <code>null</code>.
*/
public String getSource() {
return getAsString("source");
}
/**
* A table encoding a mapping from token position to line and column. This field is null if
* sources aren't available.
*
* Can return <code>null</code>.
*/
public List<List<Integer>> getTokenPosTable() {
return getListListInt("tokenPosTable");
}
/**
* The uri from which this script was loaded.
*/
public String getUri() {
return getAsString("uri");
}
}
| flutter-intellij/flutter-idea/third_party/vmServiceDrivers/org/dartlang/vm/service/element/Script.java/0 | {
"file_path": "flutter-intellij/flutter-idea/third_party/vmServiceDrivers/org/dartlang/vm/service/element/Script.java",
"repo_id": "flutter-intellij",
"token_count": 627
} | 471 |
/*
* Copyright (c) 2012, the Dart project authors.
*
* Licensed under the Eclipse Public License v1.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.eclipse.org/legal/epl-v10.html
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package org.dartlang.vm.service.logging;
/**
* The interface {@code Logger} defines the behavior of objects that can be used to receive
* information about errors. Implementations usually write this information to a file, but can also
* record the information for later use (such as during testing) or even ignore the information.
*/
public interface Logger {
/**
* Implementation of {@link Logger} that does nothing.
*/
class NullLogger implements Logger {
@Override
public void logError(String message) {
}
@Override
public void logError(String message, Throwable exception) {
}
@Override
public void logInformation(String message) {
}
@Override
public void logInformation(String message, Throwable exception) {
}
}
static final Logger NULL = new NullLogger();
/**
* Log the given message as an error.
*
* @param message an explanation of why the error occurred or what it means
*/
void logError(String message);
/**
* Log the given exception as one representing an error.
*
* @param message an explanation of why the error occurred or what it means
* @param exception the exception being logged
*/
void logError(String message, Throwable exception);
/**
* Log the given informational message.
*
* @param message an explanation of why the error occurred or what it means
*/
void logInformation(String message);
/**
* Log the given exception as one representing an informational message.
*
* @param message an explanation of why the error occurred or what it means
* @param exception the exception being logged
*/
void logInformation(String message, Throwable exception);
}
| flutter-intellij/flutter-idea/third_party/vmServiceDrivers/org/dartlang/vm/service/logging/Logger.java/0 | {
"file_path": "flutter-intellij/flutter-idea/third_party/vmServiceDrivers/org/dartlang/vm/service/logging/Logger.java",
"repo_id": "flutter-intellij",
"token_count": 629
} | 472 |
/*
* Copyright 2018 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package io.flutter.android;
import com.intellij.openapi.roots.libraries.LibraryProperties;
import com.intellij.util.xmlb.XmlSerializerUtil;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
public class AndroidModuleLibraryProperties extends LibraryProperties<AndroidModuleLibraryProperties> {
@Override
public boolean equals(Object obj) {
return false;
}
@Override
public int hashCode() {
return 0;
}
@Nullable
@Override
public AndroidModuleLibraryProperties getState() {
return this;
}
@Override
public void loadState(@NotNull AndroidModuleLibraryProperties state) {
XmlSerializerUtil.copyBean(state, this);
}
}
| flutter-intellij/flutter-studio/src/io/flutter/android/AndroidModuleLibraryProperties.java/0 | {
"file_path": "flutter-intellij/flutter-studio/src/io/flutter/android/AndroidModuleLibraryProperties.java",
"repo_id": "flutter-intellij",
"token_count": 267
} | 473 |
/*
* Copyright 2017 The Chromium Authors. All rights reserved.
* Use of this source code is governed by a BSD-style license that can be
* found in the LICENSE file.
*/
package com.android.tools.idea.tests.gui.framework;
import static com.google.common.base.Charsets.UTF_8;
import static com.google.common.io.Files.asCharSource;
import static com.intellij.openapi.util.io.FileUtil.sanitizeFileName;
import static junit.framework.TestCase.fail;
import static org.fest.reflect.core.Reflection.field;
import static org.fest.reflect.core.Reflection.method;
import static org.fest.reflect.core.Reflection.type;
import com.android.testutils.TestUtils;
import com.android.tools.idea.tests.gui.framework.aspects.AspectsAgentLogger;
import com.android.tools.idea.tests.gui.framework.fixture.FlutterFrameFixture;
import com.android.tools.idea.tests.gui.framework.fixture.FlutterWelcomeFrameFixture;
import com.android.tools.idea.tests.gui.framework.fixture.ProjectViewFixture;
import com.android.tools.idea.tests.gui.framework.fixture.WelcomeFrameFixture;
import com.android.tools.idea.tests.gui.framework.matcher.Matchers;
import com.google.common.collect.ImmutableList;
import com.intellij.ide.GeneralSettings;
import com.intellij.openapi.project.Project;
import com.intellij.openapi.project.ProjectManager;
import com.intellij.openapi.vfs.VfsUtil;
import com.intellij.openapi.vfs.VirtualFile;
import com.intellij.openapi.wm.impl.IdeFrameImpl;
import com.intellij.testGuiFramework.impl.GuiTestThread;
import com.intellij.testGuiFramework.remote.transport.RestartIdeMessage;
import io.flutter.tests.util.ProjectWrangler;
import java.awt.Component;
import java.awt.Container;
import java.awt.Dialog;
import java.awt.Frame;
import java.awt.KeyboardFocusManager;
import java.awt.Toolkit;
import java.awt.Window;
import java.awt.event.KeyEvent;
import java.beans.PropertyChangeListener;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Hashtable;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.swing.JPopupMenu;
import javax.swing.SwingUtilities;
import org.fest.swing.core.Robot;
import org.fest.swing.exception.WaitTimedOutError;
import org.fest.swing.timing.Wait;
import org.jdom.JDOMException;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import org.junit.rules.RuleChain;
import org.junit.rules.TestRule;
import org.junit.rules.Timeout;
import org.junit.runner.Description;
import org.junit.runners.model.MultipleFailureException;
import org.junit.runners.model.Statement;
/**
* A GUI test rule is used to drive GUI tests. It provides access to top-level
* UI elements, such as dialogs, IDE frame, and welcome screen (when no projects
* are open).
* <p>
* For example:
* FlutterGuiTestRule myGuiTest = new FlutterGuiTestRule();
* WizardUtils.createNewApplication(myGuiTest);
* FlutterFrameFixture ideFrame = myGuiTest.ideFrame();
* EditorFixture editor = ideFrame.getEditor();
* editor.waitUntilErrorAnalysisFinishes();
* ...
* <p>
* {@link TestRule}s can do everything that could be done previously with
* methods annotated with {@link org.junit.Before},
* {@link org.junit.After}, {@link org.junit.BeforeClass}, or
* {@link org.junit.AfterClass}, but they are more powerful, and more easily
* shared between projects and classes.
*/
@SuppressWarnings({"UnusedReturnValue", "Duplicates", "unused"})
// Adapted from com.android.tools.idea.tests.gui.framework.GuiTestRule
// Changes
// Changed field type: IdeFrameFixture -> FlutterFrameFixture
// Changed method return type: IdeFrameFixture -> FlutterFrameFixture
// Delete line containing NpwControl, which performs an update for Gradle based on finding build.gradle
// Change type ref: WelcomeFrameFixture -> FlutterWelcomeFrameFixture (but not in restartIdeIfWelcomeFrameNotShowing())
// Rewrite project open and import methods
// Add TestUtils.getWorkspaceRoot(); to setUp()
// Remove mySetNdkPath and refs to it
public class FlutterGuiTestRule implements TestRule {
/**
* Hack to solve focus issue when running with no window manager
*/
private static final boolean HAS_EXTERNAL_WINDOW_MANAGER = Toolkit.getDefaultToolkit().isFrameStateSupported(Frame.MAXIMIZED_BOTH);
private FlutterFrameFixture myIdeFrameFixture;
@Nullable private String myTestDirectory;
private final RobotTestRule myRobotTestRule = new RobotTestRule();
private final LeakCheck myLeakCheck = new LeakCheck();
/* By nesting a pair of timeouts (one around just the test, one around the entire rule chain), we ensure that Rule code executing
* before/after the test gets a chance to run, while preventing the whole rule chain from running forever.
*/
private static final int DEFAULT_TEST_TIMEOUT_MINUTES = 3;
private Timeout myInnerTimeout = new DebugFriendlyTimeout(DEFAULT_TEST_TIMEOUT_MINUTES, TimeUnit.MINUTES).withThreadDumpOnTimeout();
private Timeout myOuterTimeout = new DebugFriendlyTimeout(DEFAULT_TEST_TIMEOUT_MINUTES + 2, TimeUnit.MINUTES);
private final PropertyChangeListener myGlobalFocusListener = e -> {
Object oldValue = e.getOldValue();
if ("permanentFocusOwner".equals(e.getPropertyName()) && oldValue instanceof Component && e.getNewValue() == null) {
Window parentWindow = oldValue instanceof Window ? (Window)oldValue : SwingUtilities.getWindowAncestor((Component)oldValue);
if (parentWindow instanceof Dialog && ((Dialog)parentWindow).isModal()) {
Container parent = parentWindow.getParent();
if (parent != null && parent.isVisible()) {
parent.requestFocus();
}
}
}
};
public FlutterGuiTestRule withLeakCheck() {
myLeakCheck.setEnabled(true);
return this;
}
@NotNull
@Override
public Statement apply(final Statement base, final Description description) {
RuleChain chain = RuleChain.emptyRuleChain()
.around(new AspectsAgentLogger())
.around(new LogStartAndStop())
.around(myRobotTestRule)
.around(myOuterTimeout) // Rules should be inside this timeout when possible
.around(new IdeControl(myRobotTestRule::getRobot))
.around(new BlockReloading())
.around(new BazelUndeclaredOutputs())
.around(myLeakCheck)
.around(new FlutterGuiTestRule.IdeHandling())
.around(new ScreenshotOnFailure(myRobotTestRule::getRobot))
.around(myInnerTimeout);
// Perf logging currently writes data to the Bazel-specific TEST_UNDECLARED_OUTPUTS_DIR. Skipp logging if running outside of Bazel.
if (TestUtils.runningFromBazel()) {
chain = chain.around(new GuiPerfLogger(description));
}
return chain.apply(base, description);
}
private class IdeHandling implements TestRule {
@NotNull
@Override
public Statement apply(final Statement base, final Description description) {
return new Statement() {
@Override
public void evaluate() throws Throwable {
if (!TestUtils.runningFromBazel()) {
restartIdeIfWelcomeFrameNotShowing();
}
setUp(description.getMethodName());
List<Throwable> errors = new ArrayList<>();
try {
base.evaluate();
}
catch (MultipleFailureException e) {
errors.addAll(e.getFailures());
}
catch (Throwable e) {
errors.add(e);
}
finally {
try {
boolean hasTestPassed = errors.isEmpty();
errors.addAll(tearDown()); // shouldn't throw, but called inside a try-finally for defense in depth
if (hasTestPassed && !errors.isEmpty()) { // If we get a problem during tearDown, take a snapshot.
new ScreenshotOnFailure().failed(errors.get(0), description);
}
}
finally {
//noinspection ThrowFromFinallyBlock; assertEmpty is intended to throw here
MultipleFailureException.assertEmpty(errors);
}
}
}
};
}
}
private void restartIdeIfWelcomeFrameNotShowing() {
boolean welcomeFrameNotShowing = false;
try {
WelcomeFrameFixture.find(robot());
}
catch (WaitTimedOutError e) {
welcomeFrameNotShowing = true;
}
if (welcomeFrameNotShowing || GuiTests.windowsShowing().size() != 1) {
GuiTestThread.Companion.getClient().send(new RestartIdeMessage());
}
}
private void setUp(@Nullable String methodName) {
myTestDirectory = methodName != null ? sanitizeFileName(methodName) : null;
GeneralSettings.getInstance().setReopenLastProject(false);
GeneralSettings.getInstance().setShowTipsOnStartup(false);
GuiTests.setUpDefaultProjectCreationLocationPath(myTestDirectory);
GuiTests.setIdeSettings();
GuiTests.setUpSdks();
// Compute the workspace root before any IDE code starts messing with user.dir:
TestUtils.getWorkspaceRoot();
if (!HAS_EXTERNAL_WINDOW_MANAGER) {
KeyboardFocusManager.getCurrentKeyboardFocusManager().addPropertyChangeListener(myGlobalFocusListener);
}
}
private static ImmutableList<Throwable> thrownFromRunning(Runnable r) {
try {
r.run();
return ImmutableList.of();
}
catch (Throwable e) {
return ImmutableList.of(e);
}
}
protected void tearDownProject() {
if (!robot().finder().findAll(Matchers.byType(IdeFrameImpl.class).andIsShowing()).isEmpty()) {
ideFrame().closeProject();
}
myIdeFrameFixture = null;
}
private ImmutableList<Throwable> tearDown() {
ImmutableList.Builder<Throwable> errors = ImmutableList.builder();
errors.addAll(thrownFromRunning(this::waitForBackgroundTasks));
errors.addAll(checkForPopupMenus());
errors.addAll(checkForModalDialogs());
errors.addAll(thrownFromRunning(this::tearDownProject));
if (!HAS_EXTERNAL_WINDOW_MANAGER) {
KeyboardFocusManager.getCurrentKeyboardFocusManager().removePropertyChangeListener(myGlobalFocusListener);
}
errors.addAll(GuiTests.fatalErrorsFromIde());
fixMemLeaks();
return errors.build();
}
private List<AssertionError> checkForModalDialogs() {
List<AssertionError> errors = new ArrayList<>();
// We close all modal dialogs left over, because they block the AWT thread and could trigger a deadlock in the next test.
Dialog modalDialog;
while ((modalDialog = getActiveModalDialog()) != null) {
errors.add(new AssertionError(
String.format(
"Modal dialog %s: %s with title '%s'",
modalDialog.isShowing() ? "showing" : "not showing",
modalDialog.getClass().getName(),
modalDialog.getTitle())));
if (!modalDialog.isShowing()) break; // this assumes when the active dialog is not showing, none are showing
robot().close(modalDialog);
}
return errors;
}
private List<AssertionError> checkForPopupMenus() {
List<AssertionError> errors = new ArrayList<>();
// Close all opened popup menu items (File > New > etc) before continuing.
Collection<JPopupMenu> popupMenus = robot().finder().findAll(Matchers.byType(JPopupMenu.class).andIsShowing());
if (!popupMenus.isEmpty()) {
errors.add(new AssertionError(String.format("%d Popup Menus left open", popupMenus.size())));
for (int i = 0; i <= popupMenus.size(); i++) {
robot().pressAndReleaseKey(KeyEvent.VK_ESCAPE);
}
}
return errors;
}
// Note: this works with a cooperating window manager that returns focus properly. It does not work on bare Xvfb.
private static Dialog getActiveModalDialog() {
Window activeWindow = KeyboardFocusManager.getCurrentKeyboardFocusManager().getActiveWindow();
if (activeWindow instanceof Dialog) {
Dialog dialog = (Dialog)activeWindow;
if (dialog.getModalityType() == Dialog.ModalityType.APPLICATION_MODAL) {
return dialog;
}
}
return null;
}
private void fixMemLeaks() {
myIdeFrameFixture = null;
// Work-around for https://youtrack.jetbrains.com/issue/IDEA-153492
Class<?> keyboardManagerType = type("javax.swing.KeyboardManager").load();
Object manager = method("getCurrentManager").withReturnType(Object.class).in(keyboardManagerType).invoke();
field("componentKeyStrokeMap").ofType(Hashtable.class).in(manager).get().clear();
field("containerMap").ofType(Hashtable.class).in(manager).get().clear();
}
@NotNull
public Project openProject(@NotNull String projectDirName, boolean isImport) throws IOException, JDOMException {
ProjectWrangler wrangler = new ProjectWrangler(myTestDirectory);
VirtualFile fileToSelect = VfsUtil.findFileByIoFile(wrangler.setUpProject(projectDirName, isImport), true);
ProjectManager.getInstance().loadAndOpenProject(fileToSelect.getPath());
Wait.seconds(5).expecting("Project to be open").until(() -> ProjectManager.getInstance().getOpenProjects().length == 1);
Project project = ProjectManager.getInstance().getOpenProjects()[0];
GuiTests.waitForProjectIndexingToFinish(project);
ideFrame().updateToolbars();
return project;
}
public FlutterFrameFixture openApplication(String projectDirName) {
try {
openProject(projectDirName, false);
}
catch (JDOMException | IOException ex) {
fail();
}
ProjectViewFixture projectView = ideFrame().getProjectView();
projectView.activate();
return ideFrame();
}
public FlutterFrameFixture importApplication(String projectDirName) {
try {
openProject(projectDirName, true);
}
catch (JDOMException | IOException ex) {
fail();
}
ProjectViewFixture projectView = ideFrame().getProjectView();
projectView.activate();
return ideFrame();
}
public FlutterFrameFixture importSimpleApplication() {
return openApplication("simple_app");
}
public FlutterFrameFixture openSimpleApplication() {
return importApplication("simple_app");
}
@NotNull
protected File getMasterProjectDirPath(@NotNull String projectDirName) {
return new File(GuiTests.getTestProjectsRootDirPath(), projectDirName);
}
@NotNull
protected File getTestProjectDirPath(@NotNull String projectDirName) {
return new File(GuiTests.getProjectCreationDirPath(myTestDirectory), projectDirName);
}
public void waitForBackgroundTasks() {
GuiTests.waitForBackgroundTasks(robot());
}
public Robot robot() {
return myRobotTestRule.getRobot();
}
@NotNull
public File getProjectPath() {
return ideFrame().getProjectPath();
}
@NotNull
public File getProjectPath(@NotNull String child) {
return new File(ideFrame().getProjectPath(), child);
}
@NotNull
public String getProjectFileText(@NotNull String fileRelPath) {
try {
return asCharSource(getProjectPath(fileRelPath), UTF_8).read();
}
catch (IOException e) {
throw new RuntimeException(e);
}
}
@NotNull
public FlutterWelcomeFrameFixture welcomeFrame() {
return FlutterWelcomeFrameFixture.find(robot());
}
@NotNull
public FlutterFrameFixture ideFrame() {
if (myIdeFrameFixture == null || myIdeFrameFixture.isClosed()) {
// This call to find() creates a new IdeFrameFixture object every time. Each of these Objects creates a new gradleProjectEventListener
// and registers it with GradleSyncState. This keeps adding more and more listeners, and the new recent listeners are only updated
// with gradle State when that State changes. This means the listeners may have outdated info.
myIdeFrameFixture = FlutterFrameFixture.find(robot());
myIdeFrameFixture.requestFocusIfLost();
}
return myIdeFrameFixture;
}
public FlutterGuiTestRule withTimeout(long timeout, @NotNull TimeUnit timeUnits) {
myInnerTimeout = new Timeout(timeout, timeUnits);
myOuterTimeout = new Timeout(timeUnits.toSeconds(timeout) + 120, TimeUnit.SECONDS);
return this;
}
}
| flutter-intellij/flutter-studio/testSrc/com/android/tools/idea/tests/gui/framework/FlutterGuiTestRule.java/0 | {
"file_path": "flutter-intellij/flutter-studio/testSrc/com/android/tools/idea/tests/gui/framework/FlutterGuiTestRule.java",
"repo_id": "flutter-intellij",
"token_count": 5458
} | 474 |
cd %KOKORO_ARTIFACTS_DIR%\github\flutter-intellij-kokoro
rem Use choco to install dart so we get the latest version without having to edit this script
rem Can't find where choco puts dart-sdk
rem choco -y install dart-sdk
rem call RefreshEnv.cmd
rem Tests don't run on Windows 7
rem call tool\kokoro\test.bat
| flutter-intellij/kokoro/gcp_windows/kokoro_test.bat/0 | {
"file_path": "flutter-intellij/kokoro/gcp_windows/kokoro_test.bat",
"repo_id": "flutter-intellij",
"token_count": 101
} | 475 |
// Copyright 2017 The Chromium Authors. All rights reserved. Use of this source
// code is governed by a BSD-style license that can be found in the LICENSE file.
// Map plugin ID to JetBrains registry ID.
const Map<String, String> pluginRegistryIds = {
'io.flutter': '9212',
'io.flutter.as': '10139', // Currently unused.
};
const int cloudErrorFileMaxSize = 1000; // In bytes.
// Globals are initialized early in ProductCommand. These are used in various
// top-level functions. This is not ideal, but the "proper" solution would be
// to move nearly all the top-level functions to methods in ProductCommand.
String rootPath = '';
String lastReleaseName = '';
DateTime lastReleaseDate = DateTime.now();
int pluginCount = 0;
| flutter-intellij/tool/plugin/lib/globals.dart/0 | {
"file_path": "flutter-intellij/tool/plugin/lib/globals.dart",
"repo_id": "flutter-intellij",
"token_count": 204
} | 476 |
<!-- when updating this file also update https://github.com/flutter/.github/blob/main/CODE_OF_CONDUCT.md -->
# Code of conduct
The Flutter project expects Flutter's contributors to act professionally
and respectfully. Flutter contributors are expected to maintain the safety
and dignity of Flutter's social environments (such as GitHub and Discord).
Specifically:
* Respect people, their identities, their culture, and their work.
* Be kind. Be courteous. Be welcoming.
* Listen. Consider and acknowledge people's points before responding.
Should you experience anything that makes you feel unwelcome in Flutter's
community, please contact [[email protected]](mailto:[email protected])
or, if you prefer, directly contact someone on the project, for instance
[Hixie](mailto:[email protected]).
The Flutter project will not tolerate harassment in Flutter's
community, even outside of Flutter's public communication channels.
## Conflict resolution
When multiple contributors disagree on the direction for a particular
patch or the general direction of the project, the conflict should be
resolved by communication. The people who disagree should get
together, try to understand each other's points of view, and work to
find a design that addresses everyone's concerns.
This is usually sufficient to resolve issues. If you cannot come to an
agreement, ask for the advice of a more senior member of the project.
Be wary of agreement by attrition, where one person argues a point
repeatedly until other participants give up in the interests of moving
on. This is not conflict resolution, as it does not address everyone's
concerns. Be wary of agreement by compromise, where two good competing
solutions are merged into one mediocre solution. A conflict is
addressed when the participants agree that the final solution is
_better_ than all the conflicting proposals. Sometimes the solution is
more work than either of the proposals. [Embrace the yak
shave](https://github.com/flutter/flutter/wiki/Style-guide-for-Flutter-repo#lazy-programming).
## Questions
It's always ok to ask questions. Our systems are large, and nobody will be
an expert in all the systems. Once you find the answer, document it in
the first place you looked. That way, the next person will be brought
up to speed even quicker.
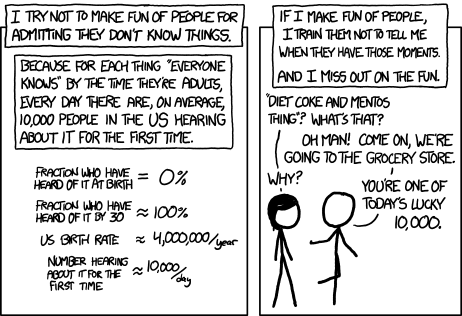
Source: _[xkcd, May 2012](https://xkcd.com/1053/)_
| flutter/CODE_OF_CONDUCT.md/0 | {
"file_path": "flutter/CODE_OF_CONDUCT.md",
"repo_id": "flutter",
"token_count": 717
} | 477 |
@ECHO off
REM Copyright 2014 The Flutter Authors. All rights reserved.
REM Use of this source code is governed by a BSD-style license that can be
REM found in the LICENSE file.
REM A script to exit caller script with the last status code.
REM This can be used with ampersand without `SETLOCAL ENABLEDELAYEDEXPANSION`.
REM
REM To use this script like `exit`, do not use with the CALL command.
REM Without CALL, this script can exit caller script, but with CALL,
REM this script returns back to caller and does not exit caller.
exit /B %ERRORLEVEL%
| flutter/bin/internal/exit_with_errorlevel.bat/0 | {
"file_path": "flutter/bin/internal/exit_with_errorlevel.bat",
"repo_id": "flutter",
"token_count": 147
} | 478 |
This directory contains tools and resources that the Flutter team uses
during the development of the framework. The tools in this directory
should not be necessary for developing Flutter applications, though of
course, they may be interesting if you are curious.
The tests in this directory are run in the `framework_tests_misc-*`
shards.
| flutter/dev/README.md/0 | {
"file_path": "flutter/dev/README.md",
"repo_id": "flutter",
"token_count": 75
} | 479 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'use_cases/use_cases.dart';
void main() {
runApp(const App());
if (kIsWeb) {
SemanticsBinding.instance.ensureSemantics();
}
}
class App extends StatelessWidget {
const App({super.key});
@override
Widget build(BuildContext context) {
final Map<String, WidgetBuilder> routes = Map<String, WidgetBuilder>.fromEntries(
useCases.map((UseCase useCase) => MapEntry<String, WidgetBuilder>(useCase.route, useCase.build)),
);
return MaterialApp(
title: 'Accessibility Assessments',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
routes: <String, WidgetBuilder>{
'/': (_) => const HomePage(),
...routes
},
);
}
}
class HomePage extends StatefulWidget {
const HomePage({super.key});
@override
State<HomePage> createState() => HomePageState();
}
class HomePageState extends State<HomePage> {
final ScrollController scrollController = ScrollController();
@override
void dispose() {
scrollController.dispose();
super.dispose();
}
Widget _buildUseCaseItem(int index, UseCase useCase) {
return Padding(
padding: const EdgeInsets.all(10),
child: Builder(
builder: (BuildContext context) {
return TextButton(
key: Key(useCase.name),
onPressed: () => Navigator.of(context).pushNamed(useCase.route),
child: Text(useCase.name),
);
}
)
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('Accessibility Assessments')),
body: Center(
child: ListView(
controller: scrollController,
children: List<Widget>.generate(
useCases.length,
(int index) => _buildUseCaseItem(index, useCases[index]),
),
),
),
);
}
}
| flutter/dev/a11y_assessments/lib/main.dart/0 | {
"file_path": "flutter/dev/a11y_assessments/lib/main.dart",
"repo_id": "flutter",
"token_count": 890
} | 480 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:a11y_assessments/main.dart';
import 'package:a11y_assessments/use_cases/use_cases.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
for (final UseCase useCase in useCases) {
testWidgets('testing accessibility guideline for ${useCase.name}', (WidgetTester tester) async {
await tester.pumpWidget(const App());
final ScrollController controller = tester.state<HomePageState>(find.byType(HomePage)).scrollController;
while (find.byKey(Key(useCase.name)).evaluate().isEmpty) {
controller.jumpTo(controller.offset + 600);
await tester.pumpAndSettle();
}
await tester.tap(find.byKey(Key(useCase.name)));
await tester.pumpAndSettle();
await expectLater(tester, meetsGuideline(textContrastGuideline));
await expectLater(tester, meetsGuideline(androidTapTargetGuideline));
await expectLater(tester, meetsGuideline(iOSTapTargetGuideline));
await expectLater(tester, meetsGuideline(labeledTapTargetGuideline));
});
}
}
| flutter/dev/a11y_assessments/test/accessibility_guideline_test.dart/0 | {
"file_path": "flutter/dev/a11y_assessments/test/accessibility_guideline_test.dart",
"repo_id": "flutter",
"token_count": 436
} | 481 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:convert';
import 'dart:io';
import 'package:flutter_driver/flutter_driver.dart';
import 'package:path/path.dart' as p;
import 'package:test/test.dart' hide TypeMatcher, isInstanceOf;
void main() {
group('semantics performance test', () {
late FlutterDriver driver;
setUpAll(() async {
// Turn off any accessibility services that may be running. The purpose of
// the test is to measure the time it takes to create the initial
// semantics tree in isolation. If accessibility services are on, the
// semantics tree gets generated during the first frame and we can't
// measure it in isolation.
final Process run = await Process.start(_adbPath(), const <String>[
'shell',
'settings',
'put',
'secure',
'enabled_accessibility_services',
'null',
]);
await run.exitCode;
driver = await FlutterDriver.connect(printCommunication: true);
});
tearDownAll(() async {
driver.close();
});
test('initial tree creation', () async {
// Let app become fully idle.
await Future<void>.delayed(const Duration(seconds: 2));
await driver.forceGC();
final Timeline timeline = await driver.traceAction(() async {
expect(
await driver.setSemantics(true),
isTrue,
reason: 'Could not toggle semantics to on because semantics were already '
'on, but the test needs to toggle semantics to measure the initial '
'semantics tree generation in isolation.'
);
});
final Iterable<TimelineEvent>? semanticsEvents = timeline.events?.where((TimelineEvent event) => event.name == 'SEMANTICS');
if (semanticsEvents?.length != 2) {
fail('Expected exactly two "SEMANTICS" events, got ${semanticsEvents?.length}:\n$semanticsEvents');
}
final Duration semanticsTreeCreation = Duration(microseconds: semanticsEvents!.last.timestampMicros! - semanticsEvents.first.timestampMicros!);
final String jsonEncoded = json.encode(<String, dynamic>{'initialSemanticsTreeCreation': semanticsTreeCreation.inMilliseconds});
File(p.join(testOutputsDirectory, 'complex_layout_semantics_perf.json')).writeAsStringSync(jsonEncoded);
}, timeout: Timeout.none);
});
}
String _adbPath() {
final String? androidHome = Platform.environment['ANDROID_HOME'] ?? Platform.environment['ANDROID_SDK_ROOT'];
if (androidHome == null) {
return 'adb';
} else {
return p.join(androidHome, 'platform-tools', 'adb');
}
}
| flutter/dev/benchmarks/complex_layout/test_driver/semantics_perf_test.dart/0 | {
"file_path": "flutter/dev/benchmarks/complex_layout/test_driver/semantics_perf_test.dart",
"repo_id": "flutter",
"token_count": 971
} | 482 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
class HeavyGridViewPage extends StatelessWidget {
const HeavyGridViewPage({super.key});
@override
Widget build(BuildContext context) {
return GridView.builder(
itemCount: 1000,
gridDelegate: const SliverGridDelegateWithFixedCrossAxisCount(crossAxisCount: 3),
itemBuilder: (BuildContext context, int index) => HeavyWidget(index),
).build(context);
}
}
class HeavyWidget extends StatelessWidget {
HeavyWidget(this.index) : super(key: ValueKey<int>(index));
final int index;
final List<int> _weight = List<int>.filled(1000000, 0);
@override
Widget build(BuildContext context) {
return SizedBox(
width: 200,
height: 200,
child: Text('$index: ${_weight.length}'),
);
}
}
| flutter/dev/benchmarks/macrobenchmarks/lib/src/heavy_grid_view.dart/0 | {
"file_path": "flutter/dev/benchmarks/macrobenchmarks/lib/src/heavy_grid_view.dart",
"repo_id": "flutter",
"token_count": 316
} | 483 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
class TextPage extends StatelessWidget {
const TextPage({super.key});
@override
Widget build(BuildContext context) {
return const Material(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
SizedBox(
width: 200,
height: 100,
child: TextField(
key: Key('basic-textfield'),
),
),
],
),
);
}
}
| flutter/dev/benchmarks/macrobenchmarks/lib/src/text.dart/0 | {
"file_path": "flutter/dev/benchmarks/macrobenchmarks/lib/src/text.dart",
"repo_id": "flutter",
"token_count": 280
} | 484 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_driver/flutter_driver.dart';
import 'package:macrobenchmarks/common.dart';
import 'util.dart';
void main() {
macroPerfTest(
'fullscreen_textfield_perf',
kFullscreenTextRouteName,
pageDelay: const Duration(seconds: 1),
driverOps: (FlutterDriver driver) async {
final SerializableFinder textfield = find.byValueKey('fullscreen-textfield');
driver.tap(textfield);
await Future<void>.delayed(const Duration(milliseconds: 5000));
},
);
}
| flutter/dev/benchmarks/macrobenchmarks/test_driver/fullscreen_textfield_perf_test.dart/0 | {
"file_path": "flutter/dev/benchmarks/macrobenchmarks/test_driver/fullscreen_textfield_perf_test.dart",
"repo_id": "flutter",
"token_count": 224
} | 485 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_driver/flutter_driver.dart';
import 'package:macrobenchmarks/common.dart';
import 'package:test/test.dart' hide TypeMatcher, isInstanceOf;
typedef DriverTestCallBack = Future<void> Function(FlutterDriver driver);
Future<void> runDriverTestForRoute(String routeName, DriverTestCallBack body) async {
final FlutterDriver driver = await FlutterDriver.connect();
// The slight initial delay avoids starting the timing during a
// period of increased load on the device. Without this delay, the
// benchmark has greater noise.
// See: https://github.com/flutter/flutter/issues/19434
await Future<void>.delayed(const Duration(milliseconds: 250));
await driver.forceGC();
final SerializableFinder scrollable = find.byValueKey(kScrollableName);
expect(scrollable, isNotNull);
final SerializableFinder button = find.byValueKey(routeName);
expect(button, isNotNull);
// -320 comes from the logical pixels for a full screen scroll for the
// smallest reference device, iPhone 4, whose physical screen dimensions are
// 960px × 640px.
const double dyScroll = -320.0;
await driver.scrollUntilVisible(scrollable, button, dyScroll: dyScroll);
await driver.tap(button);
await body(driver);
driver.close();
}
void macroPerfTest(
String testName,
String routeName, {
Duration? pageDelay,
Duration duration = const Duration(seconds: 3),
Future<void> Function(FlutterDriver driver)? driverOps,
Future<void> Function(FlutterDriver driver)? setupOps,
}) {
test(testName, () async {
late Timeline timeline;
await runDriverTestForRoute(routeName, (FlutterDriver driver) async {
if (pageDelay != null) {
// Wait for the page to load
await Future<void>.delayed(pageDelay);
}
if (setupOps != null) {
await setupOps(driver);
}
timeline = await driver.traceAction(() async {
final Future<void> durationFuture = Future<void>.delayed(duration);
if (driverOps != null) {
await driverOps(driver);
}
await durationFuture;
});
});
expect(timeline, isNotNull);
final TimelineSummary summary = TimelineSummary.summarize(timeline);
await summary.writeTimelineToFile(testName, pretty: true);
}, timeout: Timeout.none);
}
| flutter/dev/benchmarks/macrobenchmarks/test_driver/util.dart/0 | {
"file_path": "flutter/dev/benchmarks/macrobenchmarks/test_driver/util.dart",
"repo_id": "flutter",
"token_count": 778
} | 486 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:convert' show json;
import 'dart:math' as math;
double _doNormal(
{required double mean, required double stddev, required double x}) {
return (1.0 / (stddev * math.sqrt(2.0 * math.pi))) *
math.pow(math.e, -0.5 * math.pow((x - mean) / stddev, 2.0));
}
double _doMean(List<double> values) =>
values.reduce((double x, double y) => x + y) / values.length;
double _doStddev(List<double> values, double mean) {
double stddev = 0.0;
for (final double value in values) {
stddev += (value - mean) * (value - mean);
}
return math.sqrt(stddev / values.length);
}
double _doIntegral({
required double Function(double) func,
required double start,
required double stop,
required double resolution,
}) {
double result = 0.0;
while (start < stop) {
final double value = func(start);
result += resolution * value;
start += resolution;
}
return result;
}
/// Probability is defined as the probability that the mean is within the
/// [margin] of the true value.
double _doProbability({required double mean, required double stddev, required double margin}) {
return _doIntegral(
func: (double x) => _doNormal(mean: mean, stddev: stddev, x: x),
start: (1.0 - margin) * mean,
stop: (1.0 + margin) * mean,
resolution: 0.001,
);
}
/// This class knows how to format benchmark results for machine and human
/// consumption.
///
/// Example:
///
/// BenchmarkResultPrinter printer = BenchmarkResultPrinter();
/// printer.add(
/// description: 'Average frame time',
/// value: averageFrameTime,
/// unit: 'ms',
/// name: 'average_frame_time',
/// );
/// printer.printToStdout();
///
class BenchmarkResultPrinter {
final List<_BenchmarkResult> _results = <_BenchmarkResult>[];
/// Adds a benchmark result to the list of results.
///
/// [description] is a human-readable description of the result. [value] is a
/// result value. [unit] is the unit of measurement, such as "ms", "km", "h".
/// [name] is a computer-readable name of the result used as a key in the JSON
/// serialization of the results.
void addResult({ required String description, required double value, required String unit, required String name }) {
_results.add(_BenchmarkResult(description, value, unit, name));
}
/// Adds a benchmark result to the list of results and a probability of that
/// result.
///
/// The probability is calculated as the probability that the mean is +- 5% of
/// the true value.
///
/// See also [addResult].
void addResultStatistics({
required String description,
required List<double> values,
required String unit,
required String name,
}) {
final double mean = _doMean(values);
final double stddev = _doStddev(values, mean);
const double margin = 0.05;
final double probability = _doProbability(mean: mean, stddev: stddev, margin: margin);
_results.add(_BenchmarkResult(description, mean, unit, name));
_results.add(_BenchmarkResult('$description - probability margin of error $margin', probability,
'percent', '${name}_probability_5pct'));
}
/// Prints the results added via [addResult] to standard output, once as JSON
/// for computer consumption and once formatted as plain text for humans.
void printToStdout() {
// IMPORTANT: keep these values in sync with dev/devicelab/bin/tasks/microbenchmarks.dart
const String jsonStart = '================ RESULTS ================';
const String jsonEnd = '================ FORMATTED ==============';
const String jsonPrefix = ':::JSON:::';
print(jsonStart);
print('$jsonPrefix ${_printJson()}');
print(jsonEnd);
print(_printPlainText());
}
String _printJson() {
final Map<String, double> results = <String, double>{};
for (final _BenchmarkResult result in _results) {
results[result.name] = result.value;
}
return json.encode(results);
}
String _printPlainText() {
final StringBuffer buf = StringBuffer();
for (final _BenchmarkResult result in _results) {
buf.writeln('${result.description}: ${result.value.toStringAsFixed(1)} ${result.unit}');
}
return buf.toString();
}
}
class _BenchmarkResult {
_BenchmarkResult(this.description, this.value, this.unit, this.name);
/// Human-readable description of the result, e.g. "Average frame time".
final String description;
/// Result value that in agreement with [unit].
final double value;
/// Unit of measurement that is in agreement with [value].
final String unit;
/// Computer-readable name of the result.
final String name;
}
| flutter/dev/benchmarks/microbenchmarks/lib/common.dart/0 | {
"file_path": "flutter/dev/benchmarks/microbenchmarks/lib/common.dart",
"repo_id": "flutter",
"token_count": 1551
} | 487 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/foundation.dart';
import '../common.dart';
const int _kNumIterations = 10;
const int _kNumWarmUp = 100;
class Data {
Data(this.value);
final int value;
@override
String toString() => 'Data($value)';
}
List<Data> test(int length) {
return List<Data>.generate(length,
(int index) => Data(index * index));
}
Future<void> main() async {
assert(false, "Don't run benchmarks in debug mode! Use 'flutter run --release'.");
// Warm up lap
for (int i = 0; i < _kNumWarmUp; i += 1) {
await compute(test, 10);
}
final Stopwatch watch = Stopwatch();
watch.start();
for (int i = 0; i < _kNumIterations; i += 1) {
await compute(test, 1000000);
}
final int elapsedMicroseconds = watch.elapsedMicroseconds;
final BenchmarkResultPrinter printer = BenchmarkResultPrinter();
const double scale = 1000.0 / _kNumIterations;
printer.addResult(
description: 'compute',
value: elapsedMicroseconds * scale,
unit: 'ns per iteration',
name: 'compute_iteration',
);
printer.printToStdout();
}
| flutter/dev/benchmarks/microbenchmarks/lib/language/compute_bench.dart/0 | {
"file_path": "flutter/dev/benchmarks/microbenchmarks/lib/language/compute_bench.dart",
"repo_id": "flutter",
"token_count": 416
} | 488 |
#include "Generated.xcconfig"
| flutter/dev/benchmarks/test_apps/stocks/ios/Flutter/Release.xcconfig/0 | {
"file_path": "flutter/dev/benchmarks/test_apps/stocks/ios/Flutter/Release.xcconfig",
"repo_id": "flutter",
"token_count": 12
} | 489 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// THE FOLLOWING FILES WERE GENERATED BY `flutter gen-l10n`.
import 'stock_strings.dart';
/// The translations for Spanish Castilian (`es`).
class StockStringsEs extends StockStrings {
StockStringsEs([super.locale = 'es']);
@override
String get title => 'Acciones';
@override
String get market => 'MERCADO';
@override
String get portfolio => 'CARTERA';
}
| flutter/dev/benchmarks/test_apps/stocks/lib/i18n/stock_strings_es.dart/0 | {
"file_path": "flutter/dev/benchmarks/test_apps/stocks/lib/i18n/stock_strings_es.dart",
"repo_id": "flutter",
"token_count": 169
} | 490 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:stocks/main.dart' as stocks;
import 'package:stocks/stock_data.dart' as stock_data;
void main() {
stock_data.StockData.actuallyFetchData = false;
testWidgets('Search', (WidgetTester tester) async {
stocks.main(); // builds the app and schedules a frame but doesn't trigger one
await tester.pump(); // see https://github.com/flutter/flutter/issues/1865
await tester.pump(); // triggers a frame
expect(find.text('AAPL'), findsNothing);
expect(find.text('BANA'), findsNothing);
final stocks.StocksAppState app = tester.state<stocks.StocksAppState>(find.byType(stocks.StocksApp));
app.stocks.add(<List<String>>[
// "Symbol","Name","LastSale","MarketCap","IPOyear","Sector","industry","Summary Quote"
<String>['AAPL', 'Apple', '', '', '', '', '', ''],
<String>['BANA', 'Banana', '', '', '', '', '', ''],
]);
await tester.pump();
expect(find.text('AAPL'), findsOneWidget);
expect(find.text('BANA'), findsOneWidget);
await tester.tap(find.byTooltip('Search'));
// We skip a minute at a time so that each phase of the animation
// is done in two frames, the start frame and the end frame.
// There are two phases currently, so that results in three frames.
expect(await tester.pumpAndSettle(const Duration(minutes: 1)), 3);
expect(find.text('AAPL'), findsOneWidget);
expect(find.text('BANA'), findsOneWidget);
await tester.enterText(find.byType(EditableText), 'B');
await tester.pump();
expect(find.text('AAPL'), findsNothing);
expect(find.text('BANA'), findsOneWidget);
await tester.enterText(find.byType(EditableText), 'X');
await tester.pump();
expect(find.text('AAPL'), findsNothing);
expect(find.text('BANA'), findsNothing);
});
}
| flutter/dev/benchmarks/test_apps/stocks/test/search_test.dart/0 | {
"file_path": "flutter/dev/benchmarks/test_apps/stocks/test/search_test.dart",
"repo_id": "flutter",
"token_count": 717
} | 491 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:analyzer/dart/analysis/results.dart';
import 'package:analyzer/dart/ast/ast.dart';
import 'package:analyzer/dart/ast/visitor.dart';
import 'package:analyzer/dart/element/element.dart';
import 'package:analyzer/dart/element/type.dart';
import '../utils.dart';
import 'analyze.dart';
/// Verify that we use clampDouble instead of double.clamp for performance
/// reasons.
///
/// See also:
/// * https://github.com/flutter/flutter/pull/103559
/// * https://github.com/flutter/flutter/issues/103917
final AnalyzeRule noDoubleClamp = _NoDoubleClamp();
class _NoDoubleClamp implements AnalyzeRule {
final Map<ResolvedUnitResult, List<AstNode>> _errors = <ResolvedUnitResult, List<AstNode>>{};
@override
void applyTo(ResolvedUnitResult unit) {
final _DoubleClampVisitor visitor = _DoubleClampVisitor();
unit.unit.visitChildren(visitor);
final List<AstNode> violationsInUnit = visitor.clampAccessNodes;
if (violationsInUnit.isNotEmpty) {
_errors.putIfAbsent(unit, () => <AstNode>[]).addAll(violationsInUnit);
}
}
@override
void reportViolations(String workingDirectory) {
if (_errors.isEmpty) {
return;
}
foundError(<String>[
for (final MapEntry<ResolvedUnitResult, List<AstNode>> entry in _errors.entries)
for (final AstNode node in entry.value)
'${locationInFile(entry.key, node, workingDirectory)}: ${node.parent}',
'\n${bold}For performance reasons, we use a custom "clampDouble" function instead of using "double.clamp".$reset',
]);
}
@override
String toString() => 'No "double.clamp"';
}
class _DoubleClampVisitor extends RecursiveAstVisitor<void> {
final List<AstNode> clampAccessNodes = <AstNode>[];
// We don't care about directives or comments.
@override
void visitImportDirective(ImportDirective node) { }
@override
void visitExportDirective(ExportDirective node) { }
@override
void visitComment(Comment node) { }
@override
void visitSimpleIdentifier(SimpleIdentifier node) {
if (node.name != 'clamp' || node.staticElement is! MethodElement) {
return;
}
final bool isAllowed = switch (node.parent) {
// PropertyAccess matches num.clamp in tear-off form. Always prefer
// doubleClamp over tear-offs: even when all 3 operands are int literals,
// the return type doesn't get promoted to int:
// final x = 1.clamp(0, 2); // The inferred return type is int, where as:
// final f = 1.clamp;
// final y = f(0, 2) // The inferred return type is num.
PropertyAccess(
target: Expression(staticType: DartType(isDartCoreDouble: true) || DartType(isDartCoreNum: true) || DartType(isDartCoreInt: true)),
) => false,
// Expressions like `final int x = 1.clamp(0, 2);` should be allowed.
MethodInvocation(
target: Expression(staticType: DartType(isDartCoreInt: true)),
argumentList: ArgumentList(arguments: [Expression(staticType: DartType(isDartCoreInt: true)), Expression(staticType: DartType(isDartCoreInt: true))]),
) => true,
// Otherwise, disallow num.clamp() invocations.
MethodInvocation(
target: Expression(staticType: DartType(isDartCoreDouble: true) || DartType(isDartCoreNum: true) || DartType(isDartCoreInt: true)),
) => false,
_ => true,
};
if (!isAllowed) {
clampAccessNodes.add(node);
}
}
}
| flutter/dev/bots/custom_rules/no_double_clamp.dart/0 | {
"file_path": "flutter/dev/bots/custom_rules/no_double_clamp.dart",
"repo_id": "flutter",
"token_count": 1273
} | 492 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file is used by ../analyze_snippet_code_test.dart, which depends on the
// precise contents (including especially the comments) of this file.
// Examples can assume:
// bool _visible = true;
// class _Text extends Text {
// const _Text(super.text);
// const _Text.__(super.text);
// }
/// A blabla that blabla its blabla blabla blabla.
///
/// Bla blabla blabla its blabla into an blabla blabla and then blabla the
/// blabla back into the blabla blabla blabla.
///
/// Bla blabla of blabla blabla than 0.0 and 1.0, this blabla is blabla blabla
/// blabla it blabla pirates blabla the blabla into of blabla blabla. Bla the
/// blabla 0.0, the penzance blabla is blabla not blabla at all. Bla the blabla
/// 1.0, the blabla is blabla blabla blabla an blabla blabla.
///
/// {@tool snippet}
/// Bla blabla blabla some [Text] when the `_blabla` blabla blabla is true, and
/// blabla it when it is blabla:
///
/// ```dart
/// new Opacity( // error (unnecessary_new)
/// opacity: _visible ? 1.0 : 0.0,
/// child: const Text('Poor wandering ones!'),
/// )
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// Bla blabla blabla some [Text] when the `_blabla` blabla blabla is true, and
/// blabla it when it is blabla:
///
/// ```dart
/// final GlobalKey globalKey = GlobalKey();
/// ```
///
/// ```dart
/// // continuing from previous example...
/// Widget build(BuildContext context) {
/// return Opacity(
/// key: globalKey,
/// opacity: _visible ? 1.0 : 0.0,
/// child: const Text('Poor wandering ones!'),
/// );
/// }
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// Bla blabla blabla some [Text] when the `_blabla` blabla blabla is true, and
/// blabla finale blabla:
///
/// ```dart
/// Opacity(
/// opacity: _visible ? 1.0 : 0.0,
/// child: const Text('Poor wandering ones!'),
/// )
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// regular const constructor
///
/// ```dart
/// const Text('Poor wandering ones!')
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// const private constructor
/// ```dart
/// const _Text('Poor wandering ones!')
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// yet another const private constructor
/// ```dart
/// const _Text.__('Poor wandering ones!')
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// const variable
///
/// ```dart
/// const Widget text0 = Text('Poor wandering ones!');
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// more const variables
///
/// ```dart
/// const text1 = _Text('Poor wandering ones!'); // error (always_specify_types)
/// ```
/// {@end-tool}
///
/// {@tool snippet}
/// Snippet with null-safe syntax
///
/// ```dart
/// final String? bar = 'Hello'; // error (unnecessary_nullable_for_final_variable_declarations, prefer_const_declarations)
/// final int foo = null; // error (invalid_assignment, prefer_const_declarations)
/// ```
/// {@end-tool}
///
/// snippet with trailing comma
///
/// ```dart
/// const SizedBox(),
/// ```
///
/// {@tool dartpad}
/// Dartpad with null-safe syntax
///
/// ```dart
/// final GlobalKey globalKey = GlobalKey();
/// ```
///
/// ```dart
/// // not continuing from previous example...
/// Widget build(BuildContext context) {
/// final String title;
/// return Opacity(
/// key: globalKey, // error (undefined_identifier)
/// opacity: _visible ? 1.0 : 0.0,
/// child: Text(title), // error (read_potentially_unassigned_final)
/// );
/// }
/// ```
/// {@end-tool}
///
/// ```csv
/// this,is,fine
/// ```
///
/// ```dart
/// import 'dart:io'; // error (unused_import)
/// final Widget p = Placeholder(); // error (undefined_class, undefined_function)
/// ```
///
/// ```dart
/// // (e.g. in a stateful widget)
/// void initState() { // error (must_call_super, annotate_overrides)
/// widget.toString();
/// }
/// ```
///
/// ```dart
/// // not in a stateful widget
/// void initState() {
/// widget.toString(); // error (undefined_identifier)
/// }
/// ```
///
/// ```
/// error (something about backticks)
/// this must be the last error, since it aborts parsing of this file
/// ```
String? foo;
| flutter/dev/bots/test/analyze-snippet-code-test-input/known_broken_documentation.dart/0 | {
"file_path": "flutter/dev/bots/test/analyze-snippet-code-test-input/known_broken_documentation.dart",
"repo_id": "flutter",
"token_count": 1517
} | 493 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:core' hide print;
import 'dart:io' as system show exit;
import 'dart:io' hide exit;
import 'dart:math' as math;
import 'package:analyzer/dart/analysis/results.dart';
import 'package:analyzer/dart/ast/ast.dart';
import 'package:meta/meta.dart';
import 'package:path/path.dart' as path;
const Duration _quietTimeout = Duration(minutes: 10); // how long the output should be hidden between calls to printProgress before just being verbose
// If running from LUCI set to False.
final bool isLuci = Platform.environment['LUCI_CI'] == 'True';
final bool hasColor = stdout.supportsAnsiEscapes && !isLuci;
final String bold = hasColor ? '\x1B[1m' : ''; // shard titles
final String red = hasColor ? '\x1B[31m' : ''; // errors
final String green = hasColor ? '\x1B[32m' : ''; // section titles, commands
final String yellow = hasColor ? '\x1B[33m' : ''; // indications that a test was skipped (usually renders orange or brown)
final String cyan = hasColor ? '\x1B[36m' : ''; // paths
final String reverse = hasColor ? '\x1B[7m' : ''; // clocks
final String gray = hasColor ? '\x1B[30m' : ''; // subtle decorative items (usually renders as dark gray)
final String white = hasColor ? '\x1B[37m' : ''; // last log line (usually renders as light gray)
final String reset = hasColor ? '\x1B[0m' : '';
const int kESC = 0x1B;
const int kOpenSquareBracket = 0x5B;
const int kCSIParameterRangeStart = 0x30;
const int kCSIParameterRangeEnd = 0x3F;
const int kCSIIntermediateRangeStart = 0x20;
const int kCSIIntermediateRangeEnd = 0x2F;
const int kCSIFinalRangeStart = 0x40;
const int kCSIFinalRangeEnd = 0x7E;
String get redLine {
if (hasColor) {
return '$red${'━' * stdout.terminalColumns}$reset';
}
return '━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━';
}
String get clock {
final DateTime now = DateTime.now();
return '$reverse▌'
'${now.hour.toString().padLeft(2, "0")}:'
'${now.minute.toString().padLeft(2, "0")}:'
'${now.second.toString().padLeft(2, "0")}'
'▐$reset';
}
String prettyPrintDuration(Duration duration) {
String result = '';
final int minutes = duration.inMinutes;
if (minutes > 0) {
result += '${minutes}min ';
}
final int seconds = duration.inSeconds - minutes * 60;
final int milliseconds = duration.inMilliseconds - (seconds * 1000 + minutes * 60 * 1000);
result += '$seconds.${milliseconds.toString().padLeft(3, "0")}s';
return result;
}
typedef PrintCallback = void Function(Object? line);
typedef VoidCallback = void Function();
// Allow print() to be overridden, for tests.
//
// Files that import this library should not import `print` from dart:core
// and should not use dart:io's `stdout` or `stderr`.
//
// By default this hides log lines between `printProgress` calls unless a
// timeout expires or anything calls `foundError`.
//
// Also used to implement `--verbose` in test.dart.
PrintCallback print = _printQuietly;
// Called by foundError and used to implement `--abort-on-error` in test.dart.
VoidCallback? onError;
bool get hasError => _hasError;
bool _hasError = false;
List<List<String>> _errorMessages = <List<String>>[];
final List<String> _pendingLogs = <String>[];
Timer? _hideTimer; // When this is null, the output is verbose.
void foundError(List<String> messages) {
assert(messages.isNotEmpty);
// Make the error message easy to notice in the logs by
// wrapping it in a red box.
final int width = math.max(15, (hasColor ? stdout.terminalColumns : 80) - 1);
final String title = 'ERROR #${_errorMessages.length + 1}';
print('$red╔═╡$bold$title$reset$red╞═${"═" * (width - 4 - title.length)}');
for (final String message in messages.expand((String line) => line.split('\n'))) {
print('$red║$reset $message');
}
print('$red╚${"═" * width}');
// Normally, "print" actually prints to the log. To make the errors visible,
// and to include useful context, print the entire log up to this point, and
// clear it. Subsequent messages will continue to not be logged until there is
// another error.
_pendingLogs.forEach(_printLoudly);
_pendingLogs.clear();
_errorMessages.add(messages);
_hasError = true;
onError?.call();
}
@visibleForTesting
void resetErrorStatus() {
_hasError = false;
_errorMessages.clear();
_pendingLogs.clear();
_hideTimer?.cancel();
_hideTimer = null;
}
Never reportSuccessAndExit(String message) {
_hideTimer?.cancel();
_hideTimer = null;
print('$clock $message$reset');
system.exit(0);
}
Never reportErrorsAndExit(String message) {
_hideTimer?.cancel();
_hideTimer = null;
print('$clock $message$reset');
print(redLine);
print('${red}For your convenience, the error messages reported above are repeated here:$reset');
final bool printSeparators = _errorMessages.any((List<String> messages) => messages.length > 1);
if (printSeparators) {
print(' 🙙 🙛 ');
}
for (int index = 0; index < _errorMessages.length * 2 - 1; index += 1) {
if (index.isEven) {
_errorMessages[index ~/ 2].forEach(print);
} else if (printSeparators) {
print(' 🙙 🙛 ');
}
}
print(redLine);
print('You may find the errors by searching for "╡ERROR #" in the logs.');
system.exit(1);
}
void printProgress(String message) {
_pendingLogs.clear();
_hideTimer?.cancel();
_hideTimer = null;
print('$clock $message$reset');
if (hasColor) {
// This sets up a timer to switch to verbose mode when the tests take too long,
// so that if a test hangs we can see the logs.
// (This is only supported with a color terminal. When the terminal doesn't
// support colors, the scripts just print everything verbosely, that way in
// CI there's nothing hidden.)
_hideTimer = Timer(_quietTimeout, () {
_hideTimer = null;
_pendingLogs.forEach(_printLoudly);
_pendingLogs.clear();
});
}
}
final Pattern _lineBreak = RegExp(r'[\r\n]');
void _printQuietly(Object? message) {
// The point of this function is to avoid printing its output unless the timer
// has gone off in which case the function assumes verbose mode is active and
// prints everything. To show that progress is still happening though, rather
// than showing nothing at all, it instead shows the last line of output and
// keeps overwriting it. To do this in color mode, carefully measures the line
// of text ignoring color codes, which is what the parser below does.
if (_hideTimer != null) {
_pendingLogs.add(message.toString());
String line = '$message'.trimRight();
final int start = line.lastIndexOf(_lineBreak) + 1;
int index = start;
int length = 0;
while (index < line.length && length < stdout.terminalColumns) {
if (line.codeUnitAt(index) == kESC) { // 0x1B
index += 1;
if (index < line.length && line.codeUnitAt(index) == kOpenSquareBracket) { // 0x5B, [
// That was the start of a CSI sequence.
index += 1;
while (index < line.length && line.codeUnitAt(index) >= kCSIParameterRangeStart
&& line.codeUnitAt(index) <= kCSIParameterRangeEnd) { // 0x30..0x3F
index += 1; // ...parameter bytes...
}
while (index < line.length && line.codeUnitAt(index) >= kCSIIntermediateRangeStart
&& line.codeUnitAt(index) <= kCSIIntermediateRangeEnd) { // 0x20..0x2F
index += 1; // ...intermediate bytes...
}
if (index < line.length && line.codeUnitAt(index) >= kCSIFinalRangeStart
&& line.codeUnitAt(index) <= kCSIFinalRangeEnd) { // 0x40..0x7E
index += 1; // ...final byte.
}
}
} else {
index += 1;
length += 1;
}
}
line = line.substring(start, index);
if (line.isNotEmpty) {
stdout.write('\r\x1B[2K$white$line$reset');
}
} else {
_printLoudly('$message');
}
}
void _printLoudly(String message) {
if (hasColor) {
// Overwrite the last line written by _printQuietly.
stdout.writeln('\r\x1B[2K$reset${message.trimRight()}');
} else {
stdout.writeln(message);
}
}
// THE FOLLOWING CODE IS A VIOLATION OF OUR STYLE GUIDE
// BECAUSE IT INTRODUCES A VERY FLAKY RACE CONDITION
// https://github.com/flutter/flutter/wiki/Style-guide-for-Flutter-repo#never-check-if-a-port-is-available-before-using-it-never-add-timeouts-and-other-race-conditions
// DO NOT USE THE FOLLOWING FUNCTIONS
// DO NOT WRITE CODE LIKE THE FOLLOWING FUNCTIONS
// https://github.com/flutter/flutter/issues/109474
int _portCounter = 8080;
/// Finds the next available local port.
Future<int> findAvailablePortAndPossiblyCauseFlakyTests() async {
while (!await _isPortAvailable(_portCounter)) {
_portCounter += 1;
}
return _portCounter++;
}
Future<bool> _isPortAvailable(int port) async {
try {
final RawSocket socket = await RawSocket.connect('localhost', port);
socket.shutdown(SocketDirection.both);
await socket.close();
return false;
} on SocketException {
return true;
}
}
String locationInFile(ResolvedUnitResult unit, AstNode node, String workingDirectory) {
return '${path.relative(path.relative(unit.path, from: workingDirectory))}:${unit.lineInfo.getLocation(node.offset).lineNumber}';
}
| flutter/dev/bots/utils.dart/0 | {
"file_path": "flutter/dev/bots/utils.dart",
"repo_id": "flutter",
"token_count": 3476
} | 494 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:args/command_runner.dart';
import 'package:file/file.dart' show File;
import 'package:meta/meta.dart' show visibleForTesting;
import 'context.dart';
import 'git.dart';
import 'globals.dart';
import 'proto/conductor_state.pb.dart' as pb;
import 'proto/conductor_state.pbenum.dart';
import 'repository.dart';
import 'state.dart' as state_import;
const String kStateOption = 'state-file';
const String kYesFlag = 'yes';
/// Command to proceed from one [pb.ReleasePhase] to the next.
///
/// After `conductor start`, the rest of the release steps are initiated by the
/// user via `conductor next`. Thus this command's behavior is conditional upon
/// which phase of the release the user is currently in. This is implemented
/// with a switch case statement.
class NextCommand extends Command<void> {
NextCommand({
required this.checkouts,
}) {
final String defaultPath = state_import.defaultStateFilePath(checkouts.platform);
argParser.addOption(
kStateOption,
defaultsTo: defaultPath,
help: 'Path to persistent state file. Defaults to $defaultPath',
);
argParser.addFlag(
kYesFlag,
help: 'Auto-accept any confirmation prompts.',
hide: true, // primarily for integration testing
);
argParser.addFlag(
kForceFlag,
help: 'Force push when updating remote git branches.',
);
}
final Checkouts checkouts;
@override
String get name => 'next';
@override
String get description => 'Proceed to the next release phase.';
@override
Future<void> run() async {
final File stateFile = checkouts.fileSystem.file(argResults![kStateOption]);
if (!stateFile.existsSync()) {
throw ConductorException(
'No persistent state file found at ${stateFile.path}.',
);
}
final pb.ConductorState state = state_import.readStateFromFile(stateFile);
await NextContext(
autoAccept: argResults![kYesFlag] as bool,
checkouts: checkouts,
force: argResults![kForceFlag] as bool,
stateFile: stateFile,
).run(state);
}
}
/// Utility class for proceeding to the next step in a release.
///
/// Any calls to functions that cause side effects are wrapped in methods to
/// allow overriding in unit tests.
class NextContext extends Context {
const NextContext({
required this.autoAccept,
required this.force,
required super.checkouts,
required super.stateFile,
});
final bool autoAccept;
final bool force;
Future<void> run(pb.ConductorState state) async {
const List<CherrypickState> finishedStates = <CherrypickState>[
CherrypickState.COMPLETED,
CherrypickState.ABANDONED,
];
switch (state.currentPhase) {
case pb.ReleasePhase.APPLY_ENGINE_CHERRYPICKS:
final Remote upstream = Remote(
name: RemoteName.upstream,
url: state.engine.upstream.url,
);
final EngineRepository engine = EngineRepository(
checkouts,
initialRef: state.engine.workingBranch,
upstreamRemote: upstream,
previousCheckoutLocation: state.engine.checkoutPath,
);
if (!state_import.requiresEnginePR(state)) {
stdio.printStatus(
'This release has no engine cherrypicks. No Engine PR is necessary.\n',
);
break;
}
final List<pb.Cherrypick> unappliedCherrypicks = <pb.Cherrypick>[];
for (final pb.Cherrypick cherrypick in state.engine.cherrypicks) {
if (!finishedStates.contains(cherrypick.state)) {
unappliedCherrypicks.add(cherrypick);
}
}
if (unappliedCherrypicks.isEmpty) {
stdio.printStatus('All engine cherrypicks have been auto-applied by the conductor.\n');
} else {
if (unappliedCherrypicks.length == 1) {
stdio.printStatus('There was ${unappliedCherrypicks.length} cherrypick that was not auto-applied.');
} else {
stdio.printStatus('There were ${unappliedCherrypicks.length} cherrypicks that were not auto-applied.');
}
stdio.printStatus('These must be applied manually in the directory '
'${state.engine.checkoutPath} before proceeding.\n');
}
if (!autoAccept) {
final bool response = await prompt(
'Are you ready to push your engine branch to the repository '
'${state.engine.mirror.url}?',
);
if (!response) {
stdio.printError('Aborting command.');
updateState(state, stdio.logs);
return;
}
}
await pushWorkingBranch(engine, state.engine);
case pb.ReleasePhase.VERIFY_ENGINE_CI:
stdio.printStatus('You must validate post-submit CI for your engine PR and merge it');
if (!autoAccept) {
final bool response = await prompt(
'Has CI passed for the engine PR?\n\n'
'${state_import.luciConsoleLink(state.releaseChannel, 'engine')}'
);
if (!response) {
stdio.printError('Aborting command.');
updateState(state, stdio.logs);
return;
}
}
case pb.ReleasePhase.APPLY_FRAMEWORK_CHERRYPICKS:
final Remote engineUpstreamRemote = Remote(
name: RemoteName.upstream,
url: state.engine.upstream.url,
);
final EngineRepository engine = EngineRepository(
checkouts,
// We explicitly want to check out the merged version from upstream
initialRef: '${engineUpstreamRemote.name}/${state.engine.candidateBranch}',
upstreamRemote: engineUpstreamRemote,
previousCheckoutLocation: state.engine.checkoutPath,
);
final String engineRevision = await engine.reverseParse('HEAD');
final Remote upstream = Remote(
name: RemoteName.upstream,
url: state.framework.upstream.url,
);
final FrameworkRepository framework = FrameworkRepository(
checkouts,
initialRef: state.framework.workingBranch,
upstreamRemote: upstream,
previousCheckoutLocation: state.framework.checkoutPath,
);
stdio.printStatus('Writing candidate branch...');
bool needsCommit = await framework.updateCandidateBranchVersion(state.framework.candidateBranch);
if (needsCommit) {
final String revision = await framework.commit(
'Create candidate branch version ${state.framework.candidateBranch} for ${state.releaseChannel}',
addFirst: true,
);
// append to list of cherrypicks so we know a PR is required
state.framework.cherrypicks.add(pb.Cherrypick.create()
..appliedRevision = revision
..state = pb.CherrypickState.COMPLETED
);
}
stdio.printStatus('Rolling new engine hash $engineRevision to framework checkout...');
needsCommit = await framework.updateEngineRevision(engineRevision);
if (needsCommit) {
final String revision = await framework.commit(
'Update Engine revision to $engineRevision for ${state.releaseChannel} release ${state.releaseVersion}',
addFirst: true,
);
// append to list of cherrypicks so we know a PR is required
state.framework.cherrypicks.add(pb.Cherrypick.create()
..appliedRevision = revision
..state = pb.CherrypickState.COMPLETED
);
}
final List<pb.Cherrypick> unappliedCherrypicks = <pb.Cherrypick>[];
for (final pb.Cherrypick cherrypick in state.framework.cherrypicks) {
if (!finishedStates.contains(cherrypick.state)) {
unappliedCherrypicks.add(cherrypick);
}
}
if (state.framework.cherrypicks.isEmpty) {
stdio.printStatus(
'This release has no framework cherrypicks. However, a framework PR is still\n'
'required to roll engine cherrypicks.',
);
} else if (unappliedCherrypicks.isEmpty) {
stdio.printStatus('All framework cherrypicks were auto-applied by the conductor.');
} else {
if (unappliedCherrypicks.length == 1) {
stdio.printStatus('There was ${unappliedCherrypicks.length} cherrypick that was not auto-applied.',);
}
else {
stdio.printStatus('There were ${unappliedCherrypicks.length} cherrypicks that were not auto-applied.',);
}
stdio.printStatus(
'These must be applied manually in the directory '
'${state.framework.checkoutPath} before proceeding.\n',
);
}
if (!autoAccept) {
final bool response = await prompt(
'Are you ready to push your framework branch to the repository '
'${state.framework.mirror.url}?',
);
if (!response) {
stdio.printError('Aborting command.');
updateState(state, stdio.logs);
return;
}
}
await pushWorkingBranch(framework, state.framework);
case pb.ReleasePhase.PUBLISH_VERSION:
final String command = '''
tool-proxy-cli --tool_proxy=/abns/dart-eng-tool-proxy/prod-dart-eng-tool-proxy-tool-proxy.annealed-tool-proxy \\
--block_on_mpa -I flutter_release \\
:git_branch ${state.framework.candidateBranch} \\
:release_channel ${state.releaseChannel} \\
:tag ${state.releaseVersion} \\
:force false
''';
stdio.printStatus('Please ensure that you have merged your framework PR');
stdio.printStatus('and post-submit CI has finished successfully.\n');
stdio.printStatus('Run the following command, and ask a Googler');
stdio.printStatus('to review the request\n\n$command');
case pb.ReleasePhase.VERIFY_RELEASE:
stdio.printStatus(
'The current status of packaging builds can be seen at:\n'
'\t$kLuciPackagingConsoleLink',
);
if (!autoAccept) {
final bool response = await prompt(
'Have all packaging builds finished successfully and post release announcements been completed?');
if (!response) {
stdio.printError('Aborting command.');
updateState(state, stdio.logs);
return;
}
}
case pb.ReleasePhase.RELEASE_COMPLETED:
throw ConductorException('This release is finished.');
}
final ReleasePhase nextPhase = state_import.getNextPhase(state.currentPhase);
stdio.printStatus('\nUpdating phase from ${state.currentPhase} to $nextPhase...\n');
state.currentPhase = nextPhase;
stdio.printStatus(state_import.phaseInstructions(state));
updateState(state, stdio.logs);
}
/// Push the working branch to the user's mirror.
///
/// [repository] represents the actual Git repository on disk, and is used to
/// call `git push`, while [pbRepository] represents the user-specified
/// configuration for the repository, and is used to read the name of the
/// working branch and the mirror's remote name.
///
/// May throw either a [ConductorException] if the user already has a branch
/// of the same name on their mirror, or a [GitException] for any other
/// failures from the underlying git process call.
@visibleForTesting
Future<void> pushWorkingBranch(Repository repository, pb.Repository pbRepository) async {
try {
await repository.pushRef(
fromRef: 'HEAD',
// Explicitly create new branch
toRef: 'refs/heads/${pbRepository.workingBranch}',
remote: pbRepository.mirror.name,
force: force,
);
} on GitException catch (exception) {
if (exception.type == GitExceptionType.PushRejected && !force) {
throw ConductorException(
'Push failed because the working branch named '
'${pbRepository.workingBranch} already exists on your mirror. '
'Re-run this command with --force to overwrite the remote branch.\n'
'${exception.message}',
);
}
rethrow;
}
}
}
| flutter/dev/conductor/core/lib/src/next.dart/0 | {
"file_path": "flutter/dev/conductor/core/lib/src/next.dart",
"repo_id": "flutter",
"token_count": 5007
} | 495 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io';
import 'package:args/args.dart';
import 'package:file/local.dart';
import 'package:glob/glob.dart';
import 'package:path/path.dart' as path;
import 'lib/runner.dart';
Future<void> main(List<String> arguments) async {
exit(await run(arguments) ? 0 : 1);
}
// Return true if successful, false if failed.
Future<bool> run(List<String> arguments) async {
final ArgParser argParser = ArgParser(
allowTrailingOptions: false,
usageLineLength: 72,
)
..addOption(
'repeat',
defaultsTo: '1',
help: 'How many times to run each test. Set to a high value to look for flakes. If a test specifies a number of iterations, the lower of the two values is used.',
valueHelp: 'count',
)
..addOption(
'shards',
defaultsTo: '1',
help: 'How many shards to split the tests into. Used in continuous integration.',
valueHelp: 'count',
)
..addOption(
'shard-index',
defaultsTo: '0',
help: 'The current shard to run the tests with the range [0 .. shards - 1]. Used in continuous integration.',
valueHelp: 'count',
)
..addFlag(
'skip-on-fetch-failure',
help: 'Whether to skip tests that we fail to download.',
)
..addFlag(
'skip-template',
help: 'Whether to skip tests named "template.test".',
)
..addFlag(
'verbose',
help: 'Describe what is happening in detail.',
)
..addFlag(
'help',
negatable: false,
help: 'Print this help message.',
);
void printHelp() {
print('run_tests.dart [options...] path/to/file1.test path/to/file2.test...');
print('For details on the test registry format, see:');
print(' https://github.com/flutter/tests/blob/master/registry/template.test');
print('');
print(argParser.usage);
print('');
}
ArgResults parsedArguments;
try {
parsedArguments = argParser.parse(arguments);
} on ArgParserException catch (error) {
printHelp();
print('Error: ${error.message} Use --help for usage information.');
exit(1);
}
final int? repeat = int.tryParse(parsedArguments['repeat'] as String);
final bool skipOnFetchFailure = parsedArguments['skip-on-fetch-failure'] as bool;
final bool skipTemplate = parsedArguments['skip-template'] as bool;
final bool verbose = parsedArguments['verbose'] as bool;
final bool help = parsedArguments['help'] as bool;
final int? numberShards = int.tryParse(parsedArguments['shards'] as String);
final int? shardIndex = int.tryParse(parsedArguments['shard-index'] as String);
final List<File> files = parsedArguments
.rest
.expand((String path) => Glob(path).listFileSystemSync(const LocalFileSystem()))
.whereType<File>()
.where((File file) => !skipTemplate || path.basename(file.path) != 'template.test')
.toList();
if (help || repeat == null || files.isEmpty || numberShards == null || numberShards <= 0 || shardIndex == null || shardIndex < 0) {
printHelp();
if (verbose) {
if (repeat == null) {
print('Error: Could not parse repeat count ("${parsedArguments['repeat']}")');
}
if (numberShards == null) {
print('Error: Could not parse shards count ("${parsedArguments['shards']}")');
} else if (numberShards < 1) {
print('Error: The specified shards count ($numberShards) is less than 1. It must be greater than zero.');
}
if (shardIndex == null) {
print('Error: Could not parse shard index ("${parsedArguments['shard-index']}")');
} else if (shardIndex < 0) {
print('Error: The specified shard index ($shardIndex) is negative. It must be in the range [0 .. shards - 1].');
}
if (parsedArguments.rest.isEmpty) {
print('Error: No file arguments specified.');
} else if (files.isEmpty) {
print('Error: File arguments ("${parsedArguments.rest.join('", "')}") did not identify any real files.');
}
}
return help;
}
if (shardIndex > numberShards - 1) {
print(
'Error: The specified shard index ($shardIndex) is more than the specified number of shards ($numberShards). '
'It must be in the range [0 .. shards - 1].'
);
return false;
}
if (files.length < numberShards) {
print('Warning: There are more shards than tests. Some shards will not run any tests.');
}
return runTests(
repeat: repeat,
skipOnFetchFailure: skipOnFetchFailure,
verbose: verbose,
numberShards: numberShards,
shardIndex: shardIndex,
files: files,
);
}
| flutter/dev/customer_testing/run_tests.dart/0 | {
"file_path": "flutter/dev/customer_testing/run_tests.dart",
"repo_id": "flutter",
"token_count": 1733
} | 496 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This test runs `flutter test` on the `trivial_widget_test.dart` four times.
//
// The first time, the result is ignored, on the basis that it's warming the
// cache.
//
// The second time tests how long a regular test takes to run.
//
// Before the third time, a change is made to the implementation of one of the
// files that the test depends on (indirectly).
//
// Before the fourth time, a change is made to the interface in that same file.
import 'dart:async';
import 'dart:convert';
import 'dart:io';
import 'package:flutter_devicelab/framework/framework.dart';
import 'package:flutter_devicelab/framework/task_result.dart';
import 'package:flutter_devicelab/framework/utils.dart';
import 'package:path/path.dart' as path;
// Matches the output of the "test" package, e.g.: "00:01 +1 loading foo"
final RegExp testOutputPattern = RegExp(r'^[0-9][0-9]:[0-9][0-9] \+[0-9]+: (.+?) *$');
enum TestStep {
starting,
buildingFlutterTool,
runningPubGet,
testWritesFirstCarriageReturn,
testLoading,
testRunning,
testPassed,
}
Future<int> runTest({bool coverage = false, bool noPub = false}) async {
final Stopwatch clock = Stopwatch()..start();
final Process analysis = await startFlutter(
'test',
options: <String>[
if (coverage) '--coverage',
if (noPub) '--no-pub',
path.join('flutter_test', 'trivial_widget_test.dart'),
],
workingDirectory: path.join(flutterDirectory.path, 'dev', 'automated_tests'),
);
int badLines = 0;
TestStep step = TestStep.starting;
analysis.stdout.transform<String>(utf8.decoder).transform<String>(const LineSplitter()).listen((String entry) {
print('test stdout ($step): $entry');
if (step == TestStep.starting && entry == 'Building flutter tool...') {
// ignore this line
step = TestStep.buildingFlutterTool;
} else if (step == TestStep.testPassed && entry.contains('Collecting coverage information...')) {
// ignore this line
} else if (step.index < TestStep.runningPubGet.index && entry == 'Running "flutter pub get" in automated_tests...') {
// ignore this line
step = TestStep.runningPubGet;
} else if (step.index <= TestStep.testWritesFirstCarriageReturn.index && entry.trim() == '') {
// we have a blank line at the start
step = TestStep.testWritesFirstCarriageReturn;
} else {
final Match? match = testOutputPattern.matchAsPrefix(entry);
if (match == null) {
badLines += 1;
} else {
if (step.index >= TestStep.testWritesFirstCarriageReturn.index && step.index <= TestStep.testLoading.index && match.group(1)!.startsWith('loading ')) {
// first the test loads
step = TestStep.testLoading;
} else if (step.index <= TestStep.testRunning.index && match.group(1) == 'A trivial widget test') {
// then the test runs
step = TestStep.testRunning;
} else if (step.index < TestStep.testPassed.index && match.group(1) == 'All tests passed!') {
// then the test finishes
step = TestStep.testPassed;
} else {
badLines += 1;
}
}
}
});
analysis.stderr.transform<String>(utf8.decoder).transform<String>(const LineSplitter()).listen((String entry) {
print('test stderr: $entry');
badLines += 1;
});
final int result = await analysis.exitCode;
clock.stop();
if (result != 0) {
throw Exception('flutter test failed with exit code $result');
}
if (badLines > 0) {
throw Exception('flutter test rendered unexpected output ($badLines bad lines)');
}
if (step != TestStep.testPassed) {
throw Exception('flutter test did not finish (only reached step $step)');
}
print('elapsed time: ${clock.elapsedMilliseconds}ms');
return clock.elapsedMilliseconds;
}
Future<void> pubGetDependencies(List<Directory> directories) async {
for (final Directory directory in directories) {
await inDirectory<void>(directory, () async {
await flutter('pub', options: <String>['get']);
});
}
}
void main() {
task(() async {
final File nodeSourceFile = File(path.join(
flutterDirectory.path, 'packages', 'flutter', 'lib', 'src', 'foundation', 'node.dart',
));
await pubGetDependencies(<Directory>[Directory(path.join(flutterDirectory.path, 'dev', 'automated_tests')),]);
final String originalSource = await nodeSourceFile.readAsString();
try {
await runTest(noPub: true); // first number is meaningless; could have had to build the tool, run pub get, have a cache, etc
final int withoutChange = await runTest(noPub: true); // run test again with no change
await nodeSourceFile.writeAsString( // only change implementation
originalSource
.replaceAll('_owner', '_xyzzy')
);
final int implementationChange = await runTest(noPub: true); // run test again with implementation changed
await nodeSourceFile.writeAsString( // change interface as well
originalSource
.replaceAll('_owner', '_xyzzy')
.replaceAll('owner', '_owner')
.replaceAll('_xyzzy', 'owner')
);
final int interfaceChange = await runTest(noPub: true); // run test again with interface changed
// run test with coverage enabled.
final int withCoverage = await runTest(coverage: true, noPub: true);
final Map<String, dynamic> data = <String, dynamic>{
'without_change_elapsed_time_ms': withoutChange,
'implementation_change_elapsed_time_ms': implementationChange,
'interface_change_elapsed_time_ms': interfaceChange,
'with_coverage_time_ms': withCoverage,
};
return TaskResult.success(data, benchmarkScoreKeys: data.keys.toList());
} finally {
await nodeSourceFile.writeAsString(originalSource);
}
});
}
| flutter/dev/devicelab/bin/tasks/flutter_test_performance.dart/0 | {
"file_path": "flutter/dev/devicelab/bin/tasks/flutter_test_performance.dart",
"repo_id": "flutter",
"token_count": 2099
} | 497 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_devicelab/framework/apk_utils.dart';
import 'package:flutter_devicelab/framework/framework.dart';
import 'package:flutter_devicelab/framework/task_result.dart';
import 'package:flutter_devicelab/framework/utils.dart';
import 'package:path/path.dart' as path;
Future<void> main() async {
final Iterable<String> baseAabFiles = <String>[
'base/dex/classes.dex',
'base/manifest/AndroidManifest.xml',
];
final Iterable<String> flutterAabAssets = flutterAssets.map((String file) => 'base/$file');
await task(() async {
try {
await runProjectTest((FlutterProject project) async {
section('App bundle content for task bundleRelease without explicit target platform');
await inDirectory(project.rootPath, () {
return flutter('build', options: <String>[
'appbundle',
]);
});
final String releaseBundle = path.join(
project.rootPath,
'build',
'app',
'outputs',
'bundle',
'release',
'app-release.aab',
);
checkCollectionContains<String>(<String>[
...baseAabFiles,
...flutterAabAssets,
'base/lib/arm64-v8a/libapp.so',
'base/lib/arm64-v8a/libflutter.so',
'base/lib/armeabi-v7a/libapp.so',
'base/lib/armeabi-v7a/libflutter.so',
], await getFilesInAppBundle(releaseBundle));
});
await runProjectTest((FlutterProject project) async {
section('App bundle content using flavors without explicit target platform');
// Add a few flavors.
await project.addProductFlavors(<String> [
'production',
'staging',
'development',
'flavor_underscore', // https://github.com/flutter/flutter/issues/36067
]);
// Build the production flavor in release mode.
await inDirectory(project.rootPath, () {
return flutter('build', options: <String>[
'appbundle',
'--flavor',
'production',
]);
});
final String bundleFromGradlePath = path.join(
project.rootPath,
'build',
'app',
'outputs',
'bundle',
'productionRelease',
'app-production-release.aab',
);
checkCollectionContains<String>(<String>[
...baseAabFiles,
...flutterAabAssets,
'base/lib/arm64-v8a/libapp.so',
'base/lib/arm64-v8a/libflutter.so',
'base/lib/armeabi-v7a/libapp.so',
'base/lib/armeabi-v7a/libflutter.so',
], await getFilesInAppBundle(bundleFromGradlePath));
section('Build app bundle using the flutter tool - flavor: flavor_underscore');
int exitCode = await inDirectory(project.rootPath, () {
return flutter(
'build',
options: <String>[
'appbundle',
'--flavor=flavor_underscore',
'--verbose',
],
);
});
if (exitCode != 0) {
throw TaskResult.failure('flutter build appbundle command exited with code: $exitCode');
}
final String flavorUnderscoreBundlePath = path.join(
project.rootPath,
'build',
'app',
'outputs',
'bundle',
'flavor_underscoreRelease',
'app-flavor_underscore-release.aab',
);
checkCollectionContains<String>(<String>[
...baseAabFiles,
...flutterAabAssets,
'base/lib/arm64-v8a/libapp.so',
'base/lib/arm64-v8a/libflutter.so',
'base/lib/armeabi-v7a/libapp.so',
'base/lib/armeabi-v7a/libflutter.so',
], await getFilesInAppBundle(flavorUnderscoreBundlePath));
section('Build app bundle using the flutter tool - flavor: production');
exitCode = await inDirectory(project.rootPath, () {
return flutter(
'build',
options: <String>[
'appbundle',
'--flavor=production',
'--verbose',
],
);
});
if (exitCode != 0) {
throw TaskResult.failure('flutter build appbundle command exited with code: $exitCode');
}
final String productionBundlePath = path.join(
project.rootPath,
'build',
'app',
'outputs',
'bundle',
'productionRelease',
'app-production-release.aab',
);
checkCollectionContains<String>(<String>[
...baseAabFiles,
...flutterAabAssets,
'base/lib/arm64-v8a/libapp.so',
'base/lib/arm64-v8a/libflutter.so',
'base/lib/armeabi-v7a/libapp.so',
'base/lib/armeabi-v7a/libflutter.so',
], await getFilesInAppBundle(productionBundlePath));
});
await runProjectTest((FlutterProject project) async {
section('App bundle content for task bundleRelease with target platform = android-arm');
await inDirectory(project.rootPath, () {
return flutter(
'build',
options: <String>[
'appbundle',
'--target-platform=android-arm',
],
);
});
final String releaseBundle = path.join(
project.rootPath,
'build',
'app',
'outputs',
'bundle',
'release',
'app-release.aab',
);
final Iterable<String> bundleFiles = await getFilesInAppBundle(releaseBundle);
checkCollectionContains<String>(<String>[
...baseAabFiles,
...flutterAabAssets,
'base/lib/armeabi-v7a/libapp.so',
'base/lib/armeabi-v7a/libflutter.so',
], bundleFiles);
checkCollectionDoesNotContain<String>(<String>[
'base/lib/arm64-v8a/libapp.so',
'base/lib/arm64-v8a/libflutter.so',
], bundleFiles);
});
return TaskResult.success(null);
} on TaskResult catch (taskResult) {
return taskResult;
} catch (e) {
return TaskResult.failure(e.toString());
}
});
}
| flutter/dev/devicelab/bin/tasks/gradle_plugin_bundle_test.dart/0 | {
"file_path": "flutter/dev/devicelab/bin/tasks/gradle_plugin_bundle_test.dart",
"repo_id": "flutter",
"token_count": 3064
} | 498 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io';
import 'package:flutter_devicelab/framework/apk_utils.dart';
import 'package:flutter_devicelab/framework/framework.dart';
import 'package:flutter_devicelab/framework/task_result.dart';
import 'package:flutter_devicelab/framework/utils.dart';
import 'package:path/path.dart' as path;
final String gradlew = Platform.isWindows ? 'gradlew.bat' : 'gradlew';
final String gradlewExecutable = Platform.isWindows ? '.\\$gradlew' : './$gradlew';
final String fileReadWriteMode = Platform.isWindows ? 'rw-rw-rw-' : 'rw-r--r--';
final String platformLineSep = Platform.isWindows ? '\r\n': '\n';
/// Tests that the Flutter module project template works and supports
/// adding Flutter to an existing Android app.
Future<void> main() async {
await task(() async {
section('Find Java');
final String? javaHome = await findJavaHome();
if (javaHome == null) {
return TaskResult.failure('Could not find Java');
}
print('\nUsing JAVA_HOME=$javaHome');
section('Create Flutter module project');
final Directory tempDir = Directory.systemTemp.createTempSync('flutter_module_test.');
final Directory projectDir = Directory(path.join(tempDir.path, 'hello'));
try {
await inDirectory(tempDir, () async {
await flutter(
'create',
options: <String>['--org', 'io.flutter.devicelab', '--template=module', 'hello'],
);
});
section('Add read-only asset');
final File readonlyTxtAssetFile = await File(path.join(
projectDir.path,
'assets',
'read-only.txt'
))
.create(recursive: true);
if (!exists(readonlyTxtAssetFile)) {
return TaskResult.failure('Failed to create read-only asset');
}
if (!Platform.isWindows) {
await exec('chmod', <String>[
'444',
readonlyTxtAssetFile.path,
]);
}
final File pubspec = File(path.join(projectDir.path, 'pubspec.yaml'));
String content = await pubspec.readAsString();
content = content.replaceFirst(
'$platformLineSep # assets:$platformLineSep',
'$platformLineSep assets:$platformLineSep - assets/read-only.txt$platformLineSep',
);
await pubspec.writeAsString(content, flush: true);
section('Add plugins');
content = await pubspec.readAsString();
content = content.replaceFirst(
'${platformLineSep}dependencies:$platformLineSep',
'${platformLineSep}dependencies:$platformLineSep device_info: 2.0.3$platformLineSep package_info: 2.0.2$platformLineSep',
);
await pubspec.writeAsString(content, flush: true);
await inDirectory(projectDir, () async {
await flutter(
'packages',
options: <String>['get'],
);
});
section('Build Flutter module library archive');
await inDirectory(Directory(path.join(projectDir.path, '.android')), () async {
await exec(
gradlewExecutable,
<String>['flutter:assembleDebug'],
environment: <String, String>{ 'JAVA_HOME': javaHome },
);
});
final bool aarBuilt = exists(File(path.join(
projectDir.path,
'.android',
'Flutter',
'build',
'outputs',
'aar',
'flutter-debug.aar',
)));
if (!aarBuilt) {
return TaskResult.failure('Failed to build .aar');
}
section('Build ephemeral host app');
await inDirectory(projectDir, () async {
await flutter(
'build',
options: <String>['apk'],
);
});
final bool ephemeralHostApkBuilt = exists(File(path.join(
projectDir.path,
'build',
'host',
'outputs',
'apk',
'release',
'app-release.apk',
)));
if (!ephemeralHostApkBuilt) {
return TaskResult.failure('Failed to build ephemeral host .apk');
}
section('Clean build');
await inDirectory(projectDir, () async {
await flutter('clean');
});
section('Make Android host app editable');
await inDirectory(projectDir, () async {
await flutter(
'make-host-app-editable',
options: <String>['android'],
);
});
section('Build editable host app');
await inDirectory(projectDir, () async {
await flutter(
'build',
options: <String>['apk'],
);
});
final bool editableHostApkBuilt = exists(File(path.join(
projectDir.path,
'build',
'host',
'outputs',
'apk',
'release',
'app-release.apk',
)));
if (!editableHostApkBuilt) {
return TaskResult.failure('Failed to build editable host .apk');
}
section('Add to existing Android app');
final Directory hostApp = Directory(path.join(tempDir.path, 'hello_host_app'));
mkdir(hostApp);
recursiveCopy(
Directory(
path.join(
flutterDirectory.path,
'dev',
'integration_tests',
'android_custom_host_app',
),
),
hostApp,
);
copy(
File(path.join(projectDir.path, '.android', gradlew)),
hostApp,
);
copy(
File(path.join(projectDir.path, '.android', 'gradle', 'wrapper', 'gradle-wrapper.jar')),
Directory(path.join(hostApp.path, 'gradle', 'wrapper')),
);
final File analyticsOutputFile = File(path.join(tempDir.path, 'analytics.log'));
section('Build debug host APK');
await inDirectory(hostApp, () async {
if (!Platform.isWindows) {
await exec('chmod', <String>['+x', 'gradlew']);
}
await exec(gradlewExecutable,
<String>['SampleApp:assembleDebug'],
environment: <String, String>{
'JAVA_HOME': javaHome,
'FLUTTER_ANALYTICS_LOG_FILE': analyticsOutputFile.path,
},
);
});
section('Check debug APK exists');
final String debugHostApk = path.join(
hostApp.path,
'SampleApp',
'build',
'outputs',
'apk',
'debug',
'SampleApp-debug.apk',
);
if (!exists(File(debugHostApk))) {
return TaskResult.failure('Failed to build debug host APK');
}
section('Check files in debug APK');
checkCollectionContains<String>(<String>[
...flutterAssets,
...debugAssets,
...baseApkFiles,
], await getFilesInApk(debugHostApk));
section('Check debug AndroidManifest.xml');
final String androidManifestDebug = await getAndroidManifest(debugHostApk);
if (!androidManifestDebug.contains('''
<meta-data
android:name="flutterProjectType"
android:value="module" />''')
) {
return TaskResult.failure("Debug host APK doesn't contain metadata: flutterProjectType = module ");
}
final String analyticsOutput = analyticsOutputFile.readAsStringSync();
if (!analyticsOutput.contains('cd24: android')
|| !analyticsOutput.contains('cd25: true')
|| !analyticsOutput.contains('viewName: assemble')) {
return TaskResult.failure(
'Building outer app produced the following analytics: "$analyticsOutput" '
'but not the expected strings: "cd24: android", "cd25: true" and '
'"viewName: assemble"'
);
}
section('Check file access modes for read-only asset from Flutter module');
final String readonlyDebugAssetFilePath = path.joinAll(<String>[
hostApp.path,
'SampleApp',
'build',
'intermediates',
'assets',
'debug',
'flutter_assets',
'assets',
'read-only.txt',
]);
final File readonlyDebugAssetFile = File(readonlyDebugAssetFilePath);
if (!exists(readonlyDebugAssetFile)) {
return TaskResult.failure('Failed to copy read-only asset file');
}
String modes = readonlyDebugAssetFile.statSync().modeString();
print('\nread-only.txt file access modes = $modes');
if (modes.compareTo(fileReadWriteMode) != 0) {
return TaskResult.failure('Failed to make assets user-readable and writable');
}
section('Build release host APK');
await inDirectory(hostApp, () async {
await exec(gradlewExecutable,
<String>['SampleApp:assembleRelease'],
environment: <String, String>{
'JAVA_HOME': javaHome,
'FLUTTER_ANALYTICS_LOG_FILE': analyticsOutputFile.path,
},
);
});
final String releaseHostApk = path.join(
hostApp.path,
'SampleApp',
'build',
'outputs',
'apk',
'release',
'SampleApp-release-unsigned.apk',
);
if (!exists(File(releaseHostApk))) {
return TaskResult.failure('Failed to build release host APK');
}
section('Check files in release APK');
checkCollectionContains<String>(<String>[
...flutterAssets,
...baseApkFiles,
'lib/arm64-v8a/libapp.so',
'lib/arm64-v8a/libflutter.so',
'lib/armeabi-v7a/libapp.so',
'lib/armeabi-v7a/libflutter.so',
], await getFilesInApk(releaseHostApk));
section('Check release AndroidManifest.xml');
final String androidManifestRelease = await getAndroidManifest(debugHostApk);
if (!androidManifestRelease.contains('''
<meta-data
android:name="flutterProjectType"
android:value="module" />''')
) {
return TaskResult.failure("Release host APK doesn't contain metadata: flutterProjectType = module ");
}
section('Check file access modes for read-only asset from Flutter module');
final String readonlyReleaseAssetFilePath = path.joinAll(<String>[
hostApp.path,
'SampleApp',
'build',
'intermediates',
'assets',
'release',
'flutter_assets',
'assets',
'read-only.txt',
]);
final File readonlyReleaseAssetFile = File(readonlyReleaseAssetFilePath);
if (!exists(readonlyReleaseAssetFile)) {
return TaskResult.failure('Failed to copy read-only asset file');
}
modes = readonlyReleaseAssetFile.statSync().modeString();
print('\nread-only.txt file access modes = $modes');
if (modes.compareTo(fileReadWriteMode) != 0) {
return TaskResult.failure('Failed to make assets user-readable and writable');
}
return TaskResult.success(null);
} on TaskResult catch (taskResult) {
return taskResult;
} catch (e) {
return TaskResult.failure(e.toString());
} finally {
rmTree(tempDir);
}
});
}
| flutter/dev/devicelab/bin/tasks/module_custom_host_app_name_test.dart/0 | {
"file_path": "flutter/dev/devicelab/bin/tasks/module_custom_host_app_name_test.dart",
"repo_id": "flutter",
"token_count": 4728
} | 499 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_devicelab/framework/framework.dart';
import 'package:flutter_devicelab/tasks/plugin_tests.dart';
Future<void> main() async {
await task(combine(<TaskFunction>[
PluginTest('apk', <String>['-a', 'java', '--platforms=android']).call,
PluginTest('apk', <String>['-a', 'kotlin', '--platforms=android']).call,
// Test that Dart-only plugins are supported.
PluginTest('apk', <String>['--platforms=android'], dartOnlyPlugin: true).call,
// Test that FFI plugins are supported.
PluginTest('apk', <String>['--platforms=android'], template: 'plugin_ffi').call,
]));
}
| flutter/dev/devicelab/bin/tasks/plugin_test.dart/0 | {
"file_path": "flutter/dev/devicelab/bin/tasks/plugin_test.dart",
"repo_id": "flutter",
"token_count": 262
} | 500 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:args/command_runner.dart';
import '../framework/cocoon.dart';
import '../framework/metrics_center.dart';
class UploadResultsCommand extends Command<void> {
UploadResultsCommand() {
argParser.addOption('results-file', help: 'Test results JSON to upload to Cocoon.');
argParser.addOption(
'service-account-token-file',
help: 'Authentication token for uploading results.',
);
argParser.addOption('test-flaky', help: 'Flag to show whether the test is flaky: "True" or "False"');
argParser.addOption(
'git-branch',
help: '[Flutter infrastructure] Git branch of the current commit. LUCI\n'
'checkouts run in detached HEAD state, so the branch must be passed.',
);
argParser.addOption('luci-builder', help: '[Flutter infrastructure] Name of the LUCI builder being run on.');
argParser.addOption('task-name', help: '[Flutter infrastructure] Name of the task being run on.');
argParser.addOption('benchmark-tags', help: '[Flutter infrastructure] Benchmark tags to surface on Skia Perf');
argParser.addOption('test-status', help: 'Test status: Succeeded|Failed');
argParser.addOption('commit-time', help: 'Commit time in UNIX timestamp');
argParser.addOption('builder-bucket', help: '[Flutter infrastructure] Luci builder bucket the test is running in.');
}
@override
String get name => 'upload-metrics';
@override
String get description => '[Flutter infrastructure] Upload results data to Cocoon/Skia Perf';
@override
Future<void> run() async {
final String? resultsPath = argResults!['results-file'] as String?;
final String? serviceAccountTokenFile = argResults!['service-account-token-file'] as String?;
final String? testFlakyStatus = argResults!['test-flaky'] as String?;
final String? gitBranch = argResults!['git-branch'] as String?;
final String? builderName = argResults!['luci-builder'] as String?;
final String? testStatus = argResults!['test-status'] as String?;
final String? commitTime = argResults!['commit-time'] as String?;
final String? taskName = argResults!['task-name'] as String?;
final String? benchmarkTags = argResults!['benchmark-tags'] as String?;
final String? builderBucket = argResults!['builder-bucket'] as String?;
// Upload metrics to skia perf from test runner when `resultsPath` is specified.
if (resultsPath != null) {
await uploadToSkiaPerf(resultsPath, commitTime, taskName, benchmarkTags);
print('Successfully uploaded metrics to skia perf');
}
final Cocoon cocoon = Cocoon(serviceAccountTokenPath: serviceAccountTokenFile);
return cocoon.sendTaskStatus(
resultsPath: resultsPath,
isTestFlaky: testFlakyStatus == 'True',
gitBranch: gitBranch,
builderName: builderName,
testStatus: testStatus,
builderBucket: builderBucket,
);
}
}
| flutter/dev/devicelab/lib/command/upload_results.dart/0 | {
"file_path": "flutter/dev/devicelab/lib/command/upload_results.dart",
"repo_id": "flutter",
"token_count": 983
} | 501 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io';
import 'package:path/path.dart' as path;
import '../framework/task_result.dart';
import '../framework/utils.dart';
/// Run each benchmark this many times and compute average, min, max.
///
/// This must be small enough that we can do all the work in 15 minutes, the
/// devicelab deadline. Since there's four different analysis tasks, on average,
/// each can have 4 minutes. The tasks currently average a little more than a
/// minute, so that allows three runs per task.
const int _kRunsPerBenchmark = 3;
/// Path to the generated "mega gallery" app.
Directory get _megaGalleryDirectory => dir(path.join(Directory.systemTemp.path, 'mega_gallery'));
Future<TaskResult> analyzerBenchmarkTask() async {
await inDirectory<void>(flutterDirectory, () async {
rmTree(_megaGalleryDirectory);
mkdirs(_megaGalleryDirectory);
await flutter('update-packages');
await dart(<String>['dev/tools/mega_gallery.dart', '--out=${_megaGalleryDirectory.path}']);
});
final Map<String, dynamic> data = <String, dynamic>{
...(await _run(_FlutterRepoBenchmark())).asMap('flutter_repo', 'batch'),
...(await _run(_FlutterRepoBenchmark(watch: true))).asMap('flutter_repo', 'watch'),
...(await _run(_MegaGalleryBenchmark())).asMap('mega_gallery', 'batch'),
...(await _run(_MegaGalleryBenchmark(watch: true))).asMap('mega_gallery', 'watch'),
};
return TaskResult.success(data, benchmarkScoreKeys: data.keys.toList());
}
class _BenchmarkResult {
const _BenchmarkResult(this.mean, this.min, this.max);
final double mean; // seconds
final double min; // seconds
final double max; // seconds
Map<String, dynamic> asMap(String benchmark, String mode) {
return <String, dynamic>{
'${benchmark}_$mode': mean,
'${benchmark}_${mode}_minimum': min,
'${benchmark}_${mode}_maximum': max,
};
}
}
abstract class _Benchmark {
_Benchmark({this.watch = false});
final bool watch;
String get title;
Directory get directory;
List<String> get options => <String>[
'--benchmark',
if (watch) '--watch',
];
Future<double> execute(int iteration, int targetIterations) async {
section('Analyze $title ${watch ? 'with watcher' : ''} - ${iteration + 1} / $targetIterations');
final Stopwatch stopwatch = Stopwatch();
await inDirectory<void>(directory, () async {
stopwatch.start();
await flutter('analyze', options: options);
stopwatch.stop();
});
return stopwatch.elapsedMicroseconds / (1000.0 * 1000.0);
}
}
/// Times how long it takes to analyze the Flutter repository.
class _FlutterRepoBenchmark extends _Benchmark {
_FlutterRepoBenchmark({super.watch});
@override
String get title => 'Flutter repo';
@override
Directory get directory => flutterDirectory;
@override
List<String> get options {
return super.options..add('--flutter-repo');
}
}
/// Times how long it takes to analyze the generated "mega_gallery" app.
class _MegaGalleryBenchmark extends _Benchmark {
_MegaGalleryBenchmark({super.watch});
@override
String get title => 'mega gallery';
@override
Directory get directory => _megaGalleryDirectory;
}
/// Runs `benchmark` several times and reports the results.
Future<_BenchmarkResult> _run(_Benchmark benchmark) async {
final List<double> results = <double>[];
for (int i = 0; i < _kRunsPerBenchmark; i += 1) {
// Delete cached analysis results.
rmTree(dir('${Platform.environment['HOME']}/.dartServer'));
results.add(await benchmark.execute(i, _kRunsPerBenchmark));
}
results.sort();
final double sum = results.fold<double>(
0.0,
(double previousValue, double element) => previousValue + element,
);
return _BenchmarkResult(sum / results.length, results.first, results.last);
}
| flutter/dev/devicelab/lib/tasks/analysis.dart/0 | {
"file_path": "flutter/dev/devicelab/lib/tasks/analysis.dart",
"repo_id": "flutter",
"token_count": 1274
} | 502 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io' show Directory, Process;
import 'package:path/path.dart' as path;
import '../framework/devices.dart' as adb;
import '../framework/framework.dart' show TaskFunction;
import '../framework/task_result.dart' show TaskResult;
import '../framework/utils.dart' as utils;
import '../microbenchmarks.dart' as microbenchmarks;
TaskFunction runTask(adb.DeviceOperatingSystem operatingSystem) {
return () async {
adb.deviceOperatingSystem = operatingSystem;
final adb.Device device = await adb.devices.workingDevice;
await device.unlock();
final Directory appDir = utils.dir(path.join(utils.flutterDirectory.path,
'dev/benchmarks/platform_channels_benchmarks'));
final Process flutterProcess = await utils.inDirectory(appDir, () async {
final List<String> createArgs = <String>[
'--platforms',
'ios,android',
'--no-overwrite',
'-v',
'.',
];
print('\nExecuting: flutter create $createArgs $appDir');
await utils.flutter('create', options: createArgs);
final List<String> options = <String>[
'-v',
// --release doesn't work on iOS due to code signing issues
'--profile',
'--no-publish-port',
'-d',
device.deviceId,
];
return utils.startFlutter(
'run',
options: options,
);
});
final Map<String, double> results =
await microbenchmarks.readJsonResults(flutterProcess);
return TaskResult.success(results,
benchmarkScoreKeys: results.keys.toList());
};
}
| flutter/dev/devicelab/lib/tasks/platform_channels_benchmarks.dart/0 | {
"file_path": "flutter/dev/devicelab/lib/tasks/platform_channels_benchmarks.dart",
"repo_id": "flutter",
"token_count": 652
} | 503 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_devicelab/framework/runner.dart';
import 'common.dart';
void main() {
final Map<String, String> isolateParams = <String, String>{
'runFlutterConfig': 'false',
'timeoutInMinutes': '1',
};
late List<String> printLog;
void print(String s) => printLog.add(s);
group('run.dart script', () {
test('Reruns - Test passes the first time.', () async {
printLog = <String>[];
await runTasks(
<String>['smoke_test_success'],
isolateParams: isolateParams,
print: print,
logs: printLog,
);
expect(printLog.length, 2);
expect(printLog[0], 'Test passed on first attempt.');
expect(printLog[1], 'flaky: false');
});
test('Reruns - Test fails all reruns.', () async {
printLog = <String>[];
await runTasks(
<String>['smoke_test_failure'],
isolateParams: isolateParams,
print: print,
logs: printLog,
);
expect(printLog.length, 2);
expect(printLog[0], 'Consistently failed across all 3 executions.');
expect(printLog[1], 'flaky: false');
});
});
}
| flutter/dev/devicelab/test/runner_test.dart/0 | {
"file_path": "flutter/dev/devicelab/test/runner_test.dart",
"repo_id": "flutter",
"token_count": 516
} | 504 |
{
"name": "flutter",
"package": "flutter",
"author": {
"name": "The Flutter Team",
"link": "https://flutter.dev"
},
"index": "index.html",
"icon32x32": "flutter/static-assets/favicon.png",
"allowJS": true,
"ExternalURL": "https://api.flutter.dev",
"selectors": {
"#exceptions span.name a": {
"type": "Exception"
},
"h1 > span.kind-library": {
"type": "Library"
},
"h1 > span.kind-class": {
"type": "Class"
},
"h1 > span.kind-function": {
"type": "Function"
},
"h1 > span.kind-typedef": {
"type": "Type"
},
"h1 > span.kind-enum": {
"type": "Enum"
},
"h1 > span.kind-top-level-constant": {
"type": "Constant"
},
"h1 > span.kind-constant": {
"type": "Constant"
},
"h1 > span.kind-method": {
"type": "Method"
},
"h1 > span.kind-property": {
"type": "Property"
},
"h1 > span.kind-top-level-property": {
"type": "Property"
},
"h1 > span.kind-constructor": {
"type": "Constructor"
},
".callables .callable": {
"requiretext": "operator ",
"type": "Operator",
"regexp": "operator ",
"replacement": ""
}
},
"ignore": [
"ABOUT"
]
}
| flutter/dev/docs/dashing.json/0 | {
"file_path": "flutter/dev/docs/dashing.json",
"repo_id": "flutter",
"token_count": 609
} | 505 |
<script async="" defer="" src="../assets/api_survey.js"></script>
| flutter/dev/docs/survey.html/0 | {
"file_path": "flutter/dev/docs/survey.html",
"repo_id": "flutter",
"token_count": 21
} | 506 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package com.yourcompany.platforminteraction;
import java.util.HashMap;
import java.util.Map;
import java.util.List;
import java.util.ArrayList;
import java.lang.StringBuilder;
import android.graphics.Rect;
import android.os.Build;
import android.os.Bundle;
import android.util.DisplayMetrics;
import android.view.WindowManager;
import android.content.ClipboardManager;
import android.content.ClipData;
import android.content.Context;
import androidx.annotation.NonNull;
import io.flutter.embedding.engine.FlutterEngine;
import io.flutter.embedding.android.FlutterActivity;
import io.flutter.embedding.android.FlutterView;
import io.flutter.plugin.common.MethodCall;
import io.flutter.plugin.common.MethodChannel;
import io.flutter.plugin.common.MethodChannel.MethodCallHandler;
import io.flutter.plugins.GeneratedPluginRegistrant;
import android.view.accessibility.AccessibilityManager;
import android.view.accessibility.AccessibilityNodeProvider;
import android.view.accessibility.AccessibilityNodeInfo;
public class MainActivity extends FlutterActivity {
@Override
public void configureFlutterEngine(@NonNull FlutterEngine flutterEngine) {
GeneratedPluginRegistrant.registerWith(flutterEngine);
new MethodChannel(flutterEngine.getDartExecutor().getBinaryMessenger(), "semantics")
.setMethodCallHandler(new SemanticsTesterMethodHandler());
}
class SemanticsTesterMethodHandler implements MethodCallHandler {
Float mScreenDensity = 1.0f;
@Override
public void onMethodCall(MethodCall methodCall, MethodChannel.Result result) {
FlutterView flutterView = findViewById(FLUTTER_VIEW_ID);
AccessibilityNodeProvider provider = flutterView.getAccessibilityNodeProvider();
DisplayMetrics displayMetrics = new DisplayMetrics();
WindowManager wm = (WindowManager) getApplicationContext().getSystemService(Context.WINDOW_SERVICE);
wm.getDefaultDisplay().getMetrics(displayMetrics);
mScreenDensity = displayMetrics.density;
if (methodCall.method.equals("getSemanticsNode")) {
Map<String, Object> data = methodCall.arguments();
@SuppressWarnings("unchecked")
Integer id = (Integer) data.get("id");
if (id == null) {
result.error("No ID provided", "", null);
return;
}
if (provider == null) {
result.error("Semantics not enabled", "", null);
return;
}
AccessibilityNodeInfo node = provider.createAccessibilityNodeInfo(id);
result.success(convertSemantics(node, id));
return;
}
if (methodCall.method.equals("setClipboard")) {
Map<String, Object> data = methodCall.arguments();
@SuppressWarnings("unchecked")
String message = (String) data.get("message");
ClipboardManager clipboard = (ClipboardManager) getSystemService(Context.CLIPBOARD_SERVICE);
ClipData clip = ClipData.newPlainText("message", message);
clipboard.setPrimaryClip(clip);
result.success(null);
return;
}
result.notImplemented();
}
@SuppressWarnings("unchecked")
private Map<String, Object> convertSemantics(AccessibilityNodeInfo node, int id) {
if (node == null)
return null;
Map<String, Object> result = new HashMap<>();
Map<String, Object> flags = new HashMap<>();
Map<String, Object> rect = new HashMap<>();
result.put("id", id);
result.put("text", node.getText());
result.put("contentDescription", node.getContentDescription());
flags.put("isChecked", node.isChecked());
flags.put("isCheckable", node.isCheckable());
// This is not a typo.
// See: https://developer.android.com/reference/android/view/accessibility/AccessibilityNodeInfo#isDismissable()
flags.put("isDismissible", node.isDismissable());
flags.put("isEditable", node.isEditable());
flags.put("isEnabled", node.isEnabled());
flags.put("isFocusable", node.isFocusable());
flags.put("isFocused", node.isFocused());
// heading flag is only available on Android Pie or newer
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.P) {
flags.put("isHeading", node.isHeading());
}
flags.put("isPassword", node.isPassword());
flags.put("isLongClickable", node.isLongClickable());
result.put("flags", flags);
Rect nodeRect = new Rect();
node.getBoundsInScreen(nodeRect);
rect.put("left", nodeRect.left / mScreenDensity);
rect.put("top", nodeRect.top/ mScreenDensity);
rect.put("right", nodeRect.right / mScreenDensity);
rect.put("bottom", nodeRect.bottom/ mScreenDensity);
rect.put("width", nodeRect.width());
rect.put("height", nodeRect.height());
result.put("rect", rect);
result.put("className", node.getClassName());
result.put("contentDescription", node.getContentDescription());
result.put("liveRegion", node.getLiveRegion());
List<AccessibilityNodeInfo.AccessibilityAction> actionList = node.getActionList();
if (actionList.size() > 0) {
ArrayList<Integer> actions = new ArrayList<>();
for (AccessibilityNodeInfo.AccessibilityAction action : actionList) {
if (kIdByAction.containsKey(action)) {
actions.add(kIdByAction.get(action).intValue());
}
}
result.put("actions", actions);
}
return result;
}
}
// These indices need to be in sync with android_semantics_testing/lib/src/constants.dart
static final int kFocusIndex = 1 << 0;
static final int kClearFocusIndex = 1 << 1;
static final int kSelectIndex = 1 << 2;
static final int kClearSelectionIndex = 1 << 3;
static final int kClickIndex = 1 << 4;
static final int kLongClickIndex = 1 << 5;
static final int kAccessibilityFocusIndex = 1 << 6;
static final int kClearAccessibilityFocusIndex = 1 << 7;
static final int kNextAtMovementGranularityIndex = 1 << 8;
static final int kPreviousAtMovementGranularityIndex = 1 << 9;
static final int kNextHtmlElementIndex = 1 << 10;
static final int kPreviousHtmlElementIndex = 1 << 11;
static final int kScrollForwardIndex = 1 << 12;
static final int kScrollBackwardIndex = 1 << 13;
static final int kCutIndex = 1 << 14;
static final int kCopyIndex = 1 << 15;
static final int kPasteIndex = 1 << 16;
static final int kSetSelectionIndex = 1 << 17;
static final int kExpandIndex = 1 << 18;
static final int kCollapseIndex = 1 << 19;
static final int kSetText = 1 << 21;
static final Map<AccessibilityNodeInfo.AccessibilityAction, Integer> kIdByAction = new HashMap<AccessibilityNodeInfo.AccessibilityAction, Integer>() {{
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_FOCUS, kFocusIndex);
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_CLEAR_FOCUS, kClearFocusIndex);
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_SELECT, kSelectIndex);
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_CLEAR_SELECTION, kClearSelectionIndex);
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_CLICK, kClickIndex);
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_LONG_CLICK, kLongClickIndex);
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_ACCESSIBILITY_FOCUS, kAccessibilityFocusIndex);
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_CLEAR_ACCESSIBILITY_FOCUS, kClearAccessibilityFocusIndex);
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_NEXT_AT_MOVEMENT_GRANULARITY, kNextAtMovementGranularityIndex);
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_PREVIOUS_AT_MOVEMENT_GRANULARITY, kPreviousAtMovementGranularityIndex);
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_NEXT_HTML_ELEMENT, kNextHtmlElementIndex);
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_PREVIOUS_HTML_ELEMENT, kPreviousHtmlElementIndex);
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_SCROLL_FORWARD, kScrollForwardIndex);
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_SCROLL_BACKWARD, kScrollBackwardIndex);
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_CUT, kCutIndex);
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_COPY, kCopyIndex);
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_PASTE, kPasteIndex);
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_SET_SELECTION, kSetSelectionIndex);
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_EXPAND, kExpandIndex);
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_COLLAPSE, kCollapseIndex);
put(AccessibilityNodeInfo.AccessibilityAction.ACTION_SET_TEXT, kSetText);
}};
}
| flutter/dev/integration_tests/android_semantics_testing/android/app/src/main/java/com/yourcompany/platforminteraction/MainActivity.java/0 | {
"file_path": "flutter/dev/integration_tests/android_semantics_testing/android/app/src/main/java/com/yourcompany/platforminteraction/MainActivity.java",
"repo_id": "flutter",
"token_count": 3259
} | 507 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// ignore_for_file: avoid_dynamic_calls
import 'dart:convert';
import 'package:meta/meta.dart';
import 'constants.dart';
/// A semantics node created from Android accessibility information.
///
/// This object represents Android accessibility information derived from an
/// [AccessibilityNodeInfo](https://developer.android.com/reference/android/view/accessibility/AccessibilityNodeInfo)
/// object. The purpose is to verify in integration
/// tests that our semantics framework produces the correct accessibility info
/// on Android.
///
/// See also:
///
/// * [AccessibilityNodeInfo](https://developer.android.com/reference/android/view/accessibility/AccessibilityNodeInfo)
class AndroidSemanticsNode {
/// Deserializes a new [AndroidSemanticsNode] from a json map.
///
/// The structure of the JSON:
///
/// {
/// "flags": {
/// "isChecked": bool,
/// "isCheckable": bool,
/// "isEditable": bool,
/// "isEnabled": bool,
/// "isFocusable": bool,
/// "isFocused": bool,
/// "isHeading": bool,
/// "isPassword": bool,
/// "isLongClickable": bool,
/// },
/// "text": String,
/// "contentDescription": String,
/// "className": String,
/// "id": int,
/// "rect": {
/// left: int,
/// top: int,
/// right: int,
/// bottom: int,
/// },
/// actions: [
/// int,
/// ]
/// }
AndroidSemanticsNode.deserialize(String value) : _values = json.decode(value);
final dynamic _values;
final List<AndroidSemanticsNode> _children = <AndroidSemanticsNode>[];
dynamic get _flags => _values['flags'];
/// The text value of the semantics node.
///
/// This is produced by combining the value, label, and hint fields from
/// the Flutter [SemanticsNode].
String? get text => _values['text'] as String?;
/// The contentDescription of the semantics node.
///
/// This field is used for the Switch, Radio, and Checkbox widgets
/// instead of [text]. If the text property is used for these, TalkBack
/// will not read out the "checked" or "not checked" label by default.
///
/// This is produced by combining the value, label, and hint fields from
/// the Flutter [SemanticsNode].
String? get contentDescription => _values['contentDescription'] as String?;
/// The className of the semantics node.
///
/// Certain kinds of Flutter semantics are mapped to Android classes to
/// use their default semantic behavior, such as checkboxes and images.
///
/// If a more specific value isn't provided, it defaults to
/// "android.view.View".
String? get className => _values['className'] as String?;
/// The identifier for this semantics node.
int? get id => _values['id'] as int?;
/// The children of this semantics node.
List<AndroidSemanticsNode> get children => _children;
/// Whether the node is currently in a checked state.
///
/// Equivalent to [SemanticsFlag.isChecked].
bool? get isChecked => _flags['isChecked'] as bool?;
/// Whether the node can be in a checked state.
///
/// Equivalent to [SemanticsFlag.hasCheckedState]
bool? get isCheckable => _flags['isCheckable'] as bool?;
/// Whether the node is editable.
///
/// This is usually only applied to text fields, which map
/// to "android.widget.EditText".
bool? get isEditable => _flags['isEditable'] as bool?;
/// Whether the node is enabled.
bool? get isEnabled => _flags['isEnabled'] as bool?;
/// Whether the node is focusable.
bool? get isFocusable => _flags['isFocusable'] as bool?;
/// Whether the node is focused.
bool? get isFocused => _flags['isFocused'] as bool?;
/// Whether the node is considered a heading.
bool? get isHeading => _flags['isHeading'] as bool?;
/// Whether the node represents a password field.
///
/// Equivalent to [SemanticsFlag.isObscured].
bool? get isPassword => _flags['isPassword'] as bool?;
/// Whether the node is long clickable.
///
/// Equivalent to having [SemanticsAction.longPress].
bool? get isLongClickable => _flags['isLongClickable'] as bool?;
/// Gets a [Rect] which defines the position and size of the semantics node.
Rect getRect() {
final dynamic rawRect = _values['rect'];
if (rawRect == null) {
return const Rect.fromLTRB(0.0, 0.0, 0.0, 0.0);
}
return Rect.fromLTRB(
(rawRect['left']! as int).toDouble(),
(rawRect['top']! as int).toDouble(),
(rawRect['right']! as int).toDouble(),
(rawRect['bottom']! as int).toDouble(),
);
}
/// Gets a [Size] which defines the size of the semantics node.
Size getSize() {
final Rect rect = getRect();
return Size(rect.bottom - rect.top, rect.right - rect.left);
}
/// Gets a list of [AndroidSemanticsActions] which are defined for the node.
List<AndroidSemanticsAction> getActions() {
final List<int>? actions = (_values['actions'] as List<dynamic>?)?.cast<int>();
if (actions == null) {
return const <AndroidSemanticsAction>[];
}
final List<AndroidSemanticsAction> convertedActions = <AndroidSemanticsAction>[];
for (final int id in actions) {
final AndroidSemanticsAction? action = AndroidSemanticsAction.deserialize(id);
if (action != null) {
convertedActions.add(action);
}
}
return convertedActions;
}
@override
String toString() {
return _values.toString();
}
}
/// A Dart VM implementation of a rectangle.
///
/// Created to mirror the implementation of [ui.Rect].
@immutable
class Rect {
/// Creates a new rectangle.
///
/// All values are required.
const Rect.fromLTRB(this.left, this.top, this.right, this.bottom);
/// The top side of the rectangle.
final double top;
/// The left side of the rectangle.
final double left;
/// The right side of the rectangle.
final double right;
/// The bottom side of the rectangle.
final double bottom;
@override
int get hashCode => Object.hash(top, left, right, bottom);
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is Rect
&& other.top == top
&& other.left == left
&& other.right == right
&& other.bottom == bottom;
}
@override
String toString() => 'Rect.fromLTRB($left, $top, $right, $bottom)';
}
/// A Dart VM implementation of a Size.
///
/// Created to mirror the implementation [ui.Size].
@immutable
class Size {
/// Creates a new [Size] object.
const Size(this.width, this.height);
/// The width of some object.
final double width;
/// The height of some object.
final double height;
@override
int get hashCode => Object.hash(width, height);
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is Size
&& other.width == width
&& other.height == height;
}
@override
String toString() => 'Size{$width, $height}';
}
| flutter/dev/integration_tests/android_semantics_testing/lib/src/common.dart/0 | {
"file_path": "flutter/dev/integration_tests/android_semantics_testing/lib/src/common.dart",
"repo_id": "flutter",
"token_count": 2410
} | 508 |
# android\_verified\_input
Integration test that confirms that MotionEvents delivered to platform views
are verified.
| flutter/dev/integration_tests/android_verified_input/README.md/0 | {
"file_path": "flutter/dev/integration_tests/android_verified_input/README.md",
"repo_id": "flutter",
"token_count": 27
} | 509 |
# channels
Integration test of platform channels.
| flutter/dev/integration_tests/channels/README.md/0 | {
"file_path": "flutter/dev/integration_tests/channels/README.md",
"repo_id": "flutter",
"token_count": 12
} | 510 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_driver/flutter_driver.dart';
import 'package:test/test.dart' hide TypeMatcher, isInstanceOf;
Future<void> main() async {
late FlutterDriver driver;
setUpAll(() async {
driver = await FlutterDriver.connect();
});
tearDownAll(() {
driver.close();
});
// Run `run_release_test.sh` to also test release engine deferred components code. This
// drive test runs as debug and thus only tests framework side deferred components handling.
test('Install and load deferred component', () async {
final String preloadText = await driver.getText(find.byValueKey('PreloadText'));
expect(preloadText, 'preload');
final SerializableFinder fab =
find.byValueKey('FloatingActionButton');
await driver.tap(fab);
final String placeholderText = await driver.getText(find.byValueKey('PlaceholderText'));
expect(placeholderText, 'placeholder');
await driver.waitFor(find.byValueKey('DeferredWidget'));
final String deferredText = await driver.getText(find.byValueKey('DeferredWidget'));
expect(deferredText, 'DeferredWidget');
await driver.waitFor(find.byValueKey('DeferredImage'));
}, timeout: Timeout.none);
}
| flutter/dev/integration_tests/deferred_components_test/test_driver/main_test.dart/0 | {
"file_path": "flutter/dev/integration_tests/deferred_components_test/test_driver/main_test.dart",
"repo_id": "flutter",
"token_count": 412
} | 511 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
plugins {
id "com.android.application"
id "dev.flutter.flutter-gradle-plugin"
}
def localProperties = new Properties()
def localPropertiesFile = rootProject.file('local.properties')
if (localPropertiesFile.exists()) {
localPropertiesFile.withInputStream { stream ->
localProperties.load(stream)
}
}
android {
namespace "com.yourcompany.flavors"
compileSdk flutter.compileSdkVersion
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
defaultConfig {
applicationId "com.yourcompany.flavors"
minSdkVersion flutter.minSdkVersion
targetSdkVersion flutter.targetSdkVersion
versionCode 1
versionName "1.0"
}
buildTypes {
release {
signingConfig signingConfigs.debug
}
}
flavorDimensions "mode"
productFlavors {
free {}
paid {}
}
}
flutter {
source '../..'
}
| flutter/dev/integration_tests/flavors/android/app/build.gradle/0 | {
"file_path": "flutter/dev/integration_tests/flavors/android/app/build.gradle",
"repo_id": "flutter",
"token_count": 446
} | 512 |
// This xcconfig used for both the paid and free flavor, but the plugins are not different between
// them. Pick one.
#include? "Pods/Target Support Files/Pods-Free App/Pods-Free App.debug free.xcconfig"
#include "Generated.xcconfig"
| flutter/dev/integration_tests/flavors/ios/Flutter/Debug.xcconfig/0 | {
"file_path": "flutter/dev/integration_tests/flavors/ios/Flutter/Debug.xcconfig",
"repo_id": "flutter",
"token_count": 74
} | 513 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
export 'animation_demo.dart';
export 'calculator_demo.dart';
export 'colors_demo.dart';
export 'contacts_demo.dart';
export 'cupertino/cupertino.dart';
export 'fortnightly/fortnightly.dart';
export 'images_demo.dart';
export 'material/material.dart';
export 'pesto_demo.dart';
export 'shrine_demo.dart';
export 'transformations/transformations_demo.dart';
export 'typography_demo.dart';
export 'video_demo.dart';
| flutter/dev/integration_tests/flutter_gallery/lib/demo/all.dart/0 | {
"file_path": "flutter/dev/integration_tests/flutter_gallery/lib/demo/all.dart",
"repo_id": "flutter",
"token_count": 203
} | 514 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math' show Random;
import 'package:flutter/cupertino.dart';
import '../../gallery/demo.dart';
class CupertinoRefreshControlDemo extends StatefulWidget {
const CupertinoRefreshControlDemo({super.key});
static const String routeName = '/cupertino/refresh';
@override
State<CupertinoRefreshControlDemo> createState() => _CupertinoRefreshControlDemoState();
}
class _CupertinoRefreshControlDemoState extends State<CupertinoRefreshControlDemo> {
late List<List<String>> randomizedContacts;
@override
void initState() {
super.initState();
repopulateList();
}
void repopulateList() {
final Random random = Random();
randomizedContacts = List<List<String>>.generate(
100,
(int index) {
return contacts[random.nextInt(contacts.length)]
// Randomly adds a telephone icon next to the contact or not.
..add(random.nextBool().toString());
},
);
}
@override
Widget build(BuildContext context) {
return DefaultTextStyle(
style: CupertinoTheme.of(context).textTheme.textStyle,
child: CupertinoPageScaffold(
backgroundColor: CupertinoColors.systemGroupedBackground,
child: CustomScrollView(
// If left unspecified, the [CustomScrollView] appends an
// [AlwaysScrollableScrollPhysics]. Behind the scene, the ScrollableState
// will attach that [AlwaysScrollableScrollPhysics] to the output of
// [ScrollConfiguration.of] which will be a [ClampingScrollPhysics]
// on Android.
// To demonstrate the iOS behavior in this demo and to ensure that the list
// always scrolls, we specifically use a [BouncingScrollPhysics] combined
// with a [AlwaysScrollableScrollPhysics]
physics: const BouncingScrollPhysics(parent: AlwaysScrollableScrollPhysics()),
slivers: <Widget>[
CupertinoSliverNavigationBar(
largeTitle: const Text('Refresh'),
// We're specifying a back label here because the previous page
// is a Material page. CupertinoPageRoutes could auto-populate
// these back labels.
previousPageTitle: 'Cupertino',
trailing: CupertinoDemoDocumentationButton(CupertinoRefreshControlDemo.routeName),
),
CupertinoSliverRefreshControl(
onRefresh: () {
return Future<void>.delayed(const Duration(seconds: 2))
..then((_) {
if (mounted) {
setState(() => repopulateList());
}
});
},
),
SliverSafeArea(
top: false, // Top safe area is consumed by the navigation bar.
sliver: SliverList(
delegate: SliverChildBuilderDelegate(
(BuildContext context, int index) {
return _ListItem(
name: randomizedContacts[index][0],
place: randomizedContacts[index][1],
date: randomizedContacts[index][2],
called: randomizedContacts[index][3] == 'true',
);
},
childCount: 20,
),
),
),
],
),
),
);
}
}
List<List<String>> contacts = <List<String>>[
<String>['George Washington', 'Westmoreland County', ' 4/30/1789'],
<String>['John Adams', 'Braintree', ' 3/4/1797'],
<String>['Thomas Jefferson', 'Shadwell', ' 3/4/1801'],
<String>['James Madison', 'Port Conway', ' 3/4/1809'],
<String>['James Monroe', 'Monroe Hall', ' 3/4/1817'],
<String>['Andrew Jackson', 'Waxhaws Region South/North', ' 3/4/1829'],
<String>['John Quincy Adams', 'Braintree', ' 3/4/1825'],
<String>['William Henry Harrison', 'Charles City County', ' 3/4/1841'],
<String>['Martin Van Buren', 'Kinderhook New', ' 3/4/1837'],
<String>['Zachary Taylor', 'Barboursville', ' 3/4/1849'],
<String>['John Tyler', 'Charles City County', ' 4/4/1841'],
<String>['James Buchanan', 'Cove Gap', ' 3/4/1857'],
<String>['James K. Polk', 'Pineville North', ' 3/4/1845'],
<String>['Millard Fillmore', 'Summerhill New', '7/9/1850'],
<String>['Franklin Pierce', 'Hillsborough New', ' 3/4/1853'],
<String>['Andrew Johnson', 'Raleigh North', ' 4/15/1865'],
<String>['Abraham Lincoln', 'Sinking Spring', ' 3/4/1861'],
<String>['Ulysses S. Grant', 'Point Pleasant', ' 3/4/1869'],
<String>['Rutherford B. Hayes', 'Delaware', ' 3/4/1877'],
<String>['Chester A. Arthur', 'Fairfield', ' 9/19/1881'],
<String>['James A. Garfield', 'Moreland Hills', ' 3/4/1881'],
<String>['Benjamin Harrison', 'North Bend', ' 3/4/1889'],
<String>['Grover Cleveland', 'Caldwell New', ' 3/4/1885'],
<String>['William McKinley', 'Niles', ' 3/4/1897'],
<String>['Woodrow Wilson', 'Staunton', ' 3/4/1913'],
<String>['William H. Taft', 'Cincinnati', ' 3/4/1909'],
<String>['Theodore Roosevelt', 'New York City New', ' 9/14/1901'],
<String>['Warren G. Harding', 'Blooming Grove', ' 3/4/1921'],
<String>['Calvin Coolidge', 'Plymouth', '8/2/1923'],
<String>['Herbert Hoover', 'West Branch', ' 3/4/1929'],
<String>['Franklin D. Roosevelt', 'Hyde Park New', ' 3/4/1933'],
<String>['Harry S. Truman', 'Lamar', ' 4/12/1945'],
<String>['Dwight D. Eisenhower', 'Denison', ' 1/20/1953'],
<String>['Lyndon B. Johnson', 'Stonewall', '11/22/1963'],
<String>['Ronald Reagan', 'Tampico', ' 1/20/1981'],
<String>['Richard Nixon', 'Yorba Linda', ' 1/20/1969'],
<String>['Gerald Ford', 'Omaha', 'August 9/1974'],
<String>['John F. Kennedy', 'Brookline', ' 1/20/1961'],
<String>['George H. W. Bush', 'Milton', ' 1/20/1989'],
<String>['Jimmy Carter', 'Plains', ' 1/20/1977'],
<String>['George W. Bush', 'New Haven', ' 1/20, 2001'],
<String>['Bill Clinton', 'Hope', ' 1/20/1993'],
<String>['Barack Obama', 'Honolulu', ' 1/20/2009'],
<String>['Donald J. Trump', 'New York City', ' 1/20/2017'],
];
class _ListItem extends StatelessWidget {
const _ListItem({
this.name,
this.place,
this.date,
this.called,
});
final String? name;
final String? place;
final String? date;
final bool? called;
@override
Widget build(BuildContext context) {
return Container(
color: CupertinoDynamicColor.resolve(CupertinoColors.systemBackground, context),
height: 60.0,
padding: const EdgeInsets.only(top: 9.0),
child: Row(
children: <Widget>[
SizedBox(
width: 38.0,
child: called!
? Align(
alignment: Alignment.topCenter,
child: Icon(
CupertinoIcons.phone_solid,
color: CupertinoColors.inactiveGray.resolveFrom(context),
size: 18.0,
),
)
: null,
),
Expanded(
child: Container(
decoration: const BoxDecoration(
border: Border(
bottom: BorderSide(color: Color(0xFFBCBBC1), width: 0.0),
),
),
padding: const EdgeInsets.only(left: 1.0, bottom: 9.0, right: 10.0),
child: Row(
children: <Widget>[
Expanded(
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
Text(
name!,
maxLines: 1,
overflow: TextOverflow.ellipsis,
style: const TextStyle(
fontWeight: FontWeight.w600,
letterSpacing: -0.18,
),
),
Text(
place!,
maxLines: 1,
overflow: TextOverflow.ellipsis,
style: TextStyle(
fontSize: 15.0,
letterSpacing: -0.24,
color: CupertinoColors.inactiveGray.resolveFrom(context),
),
),
],
),
),
Text(
date!,
style: TextStyle(
color: CupertinoColors.inactiveGray.resolveFrom(context),
fontSize: 15.0,
letterSpacing: -0.41,
),
),
Padding(
padding: const EdgeInsets.only(left: 9.0),
child: Icon(
CupertinoIcons.info,
color: CupertinoTheme.of(context).primaryColor,
),
),
],
),
),
),
],
),
);
}
}
| flutter/dev/integration_tests/flutter_gallery/lib/demo/cupertino/cupertino_refresh_demo.dart/0 | {
"file_path": "flutter/dev/integration_tests/flutter_gallery/lib/demo/cupertino/cupertino_refresh_demo.dart",
"repo_id": "flutter",
"token_count": 4609
} | 515 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:intl/intl.dart';
import '../../gallery/demo.dart';
class _InputDropdown extends StatelessWidget {
const _InputDropdown({
this.labelText,
this.valueText,
this.valueStyle,
this.onPressed,
});
final String? labelText;
final String? valueText;
final TextStyle? valueStyle;
final VoidCallback? onPressed;
@override
Widget build(BuildContext context) {
return InkWell(
onTap: onPressed,
child: InputDecorator(
decoration: InputDecoration(
labelText: labelText,
),
baseStyle: valueStyle,
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Text(valueText!, style: valueStyle),
Icon(Icons.arrow_drop_down,
color: Theme.of(context).brightness == Brightness.light ? Colors.grey.shade700 : Colors.white70,
),
],
),
),
);
}
}
class _DateTimePicker extends StatelessWidget {
const _DateTimePicker({
this.labelText,
this.selectedDate,
this.selectedTime,
this.selectDate,
this.selectTime,
});
final String? labelText;
final DateTime? selectedDate;
final TimeOfDay? selectedTime;
final ValueChanged<DateTime>? selectDate;
final ValueChanged<TimeOfDay>? selectTime;
Future<void> _selectDate(BuildContext context) async {
final DateTime? picked = await showDatePicker(
context: context,
initialDate: selectedDate,
firstDate: DateTime(2015, 8),
lastDate: DateTime(2101),
);
if (picked != null && picked != selectedDate) {
selectDate!(picked);
}
}
Future<void> _selectTime(BuildContext context) async {
final TimeOfDay? picked = await showTimePicker(
context: context,
initialTime: selectedTime!,
);
if (picked != null && picked != selectedTime) {
selectTime!(picked);
}
}
@override
Widget build(BuildContext context) {
final TextStyle? valueStyle = Theme.of(context).textTheme.titleLarge;
return Row(
crossAxisAlignment: CrossAxisAlignment.end,
children: <Widget>[
Expanded(
flex: 4,
child: _InputDropdown(
labelText: labelText,
valueText: DateFormat.yMMMd().format(selectedDate!),
valueStyle: valueStyle,
onPressed: () { _selectDate(context); },
),
),
const SizedBox(width: 12.0),
Expanded(
flex: 3,
child: _InputDropdown(
valueText: selectedTime!.format(context),
valueStyle: valueStyle,
onPressed: () { _selectTime(context); },
),
),
],
);
}
}
class DateAndTimePickerDemo extends StatefulWidget {
const DateAndTimePickerDemo({super.key});
static const String routeName = '/material/date-and-time-pickers';
@override
State<DateAndTimePickerDemo> createState() => _DateAndTimePickerDemoState();
}
class _DateAndTimePickerDemoState extends State<DateAndTimePickerDemo> {
DateTime? _fromDate = DateTime.now();
TimeOfDay _fromTime = const TimeOfDay(hour: 7, minute: 28);
DateTime? _toDate = DateTime.now();
TimeOfDay _toTime = const TimeOfDay(hour: 8, minute: 28);
final List<String> _allActivities = <String>['hiking', 'swimming', 'boating', 'fishing'];
String? _activity = 'fishing';
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Date and time pickers'),
actions: <Widget>[MaterialDemoDocumentationButton(DateAndTimePickerDemo.routeName)],
),
body: DropdownButtonHideUnderline(
child: SafeArea(
top: false,
bottom: false,
child: ListView(
padding: const EdgeInsets.all(16.0),
children: <Widget>[
TextField(
enabled: true,
decoration: const InputDecoration(
labelText: 'Event name',
border: OutlineInputBorder(),
),
style: Theme.of(context).textTheme.headlineMedium,
),
TextField(
decoration: const InputDecoration(
labelText: 'Location',
),
style: Theme.of(context).textTheme.headlineMedium!.copyWith(fontSize: 20.0),
),
_DateTimePicker(
labelText: 'From',
selectedDate: _fromDate,
selectedTime: _fromTime,
selectDate: (DateTime date) {
setState(() {
_fromDate = date;
});
},
selectTime: (TimeOfDay time) {
setState(() {
_fromTime = time;
});
},
),
_DateTimePicker(
labelText: 'To',
selectedDate: _toDate,
selectedTime: _toTime,
selectDate: (DateTime date) {
setState(() {
_toDate = date;
});
},
selectTime: (TimeOfDay time) {
setState(() {
_toTime = time;
});
},
),
const SizedBox(height: 8.0),
InputDecorator(
decoration: const InputDecoration(
labelText: 'Activity',
hintText: 'Choose an activity',
contentPadding: EdgeInsets.zero,
),
isEmpty: _activity == null,
child: DropdownButton<String>(
value: _activity,
onChanged: (String? newValue) {
setState(() {
_activity = newValue;
});
},
items: _allActivities.map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList(),
),
),
],
),
),
),
);
}
}
| flutter/dev/integration_tests/flutter_gallery/lib/demo/material/date_and_time_picker_demo.dart/0 | {
"file_path": "flutter/dev/integration_tests/flutter_gallery/lib/demo/material/date_and_time_picker_demo.dart",
"repo_id": "flutter",
"token_count": 3219
} | 516 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import '../../gallery/demo.dart';
class PersistentBottomSheetDemo extends StatefulWidget {
const PersistentBottomSheetDemo({super.key});
static const String routeName = '/material/persistent-bottom-sheet';
@override
State<PersistentBottomSheetDemo> createState() => _PersistentBottomSheetDemoState();
}
class _PersistentBottomSheetDemoState extends State<PersistentBottomSheetDemo> {
final GlobalKey<ScaffoldState> _scaffoldKey = GlobalKey<ScaffoldState>();
VoidCallback? _showBottomSheetCallback;
@override
void initState() {
super.initState();
_showBottomSheetCallback = _showBottomSheet;
}
void _showBottomSheet() {
setState(() { // disable the button
_showBottomSheetCallback = null;
});
_scaffoldKey.currentState!.showBottomSheet((BuildContext context) {
final ThemeData themeData = Theme.of(context);
return Container(
decoration: BoxDecoration(
border: Border(top: BorderSide(color: themeData.disabledColor))
),
child: Padding(
padding: const EdgeInsets.all(32.0),
child: Text('This is a Material persistent bottom sheet. Drag downwards to dismiss it.',
textAlign: TextAlign.center,
style: TextStyle(
color: themeData.colorScheme.secondary,
fontSize: 24.0,
),
),
),
);
})
.closed.whenComplete(() {
if (mounted) {
setState(() { // re-enable the button
_showBottomSheetCallback = _showBottomSheet;
});
}
});
}
void _showMessage() {
showDialog<void>(
context: context,
builder: (BuildContext context) {
return AlertDialog(
content: const Text('You tapped the floating action button.'),
actions: <Widget>[
TextButton(
onPressed: () {
Navigator.pop(context);
},
child: const Text('OK'),
),
],
);
},
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
key: _scaffoldKey,
appBar: AppBar(
title: const Text('Persistent bottom sheet'),
actions: <Widget>[
MaterialDemoDocumentationButton(PersistentBottomSheetDemo.routeName),
],
),
floatingActionButton: FloatingActionButton(
onPressed: _showMessage,
backgroundColor: Colors.redAccent,
child: const Icon(
Icons.add,
semanticLabel: 'Add',
),
),
body: Center(
child: ElevatedButton(
onPressed: _showBottomSheetCallback,
child: const Text('SHOW BOTTOM SHEET'),
),
),
);
}
}
| flutter/dev/integration_tests/flutter_gallery/lib/demo/material/persistent_bottom_sheet_demo.dart/0 | {
"file_path": "flutter/dev/integration_tests/flutter_gallery/lib/demo/material/persistent_bottom_sheet_demo.dart",
"repo_id": "flutter",
"token_count": 1240
} | 517 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
const Color kShrinePink50 = Color(0xFFFEEAE6);
const Color kShrinePink100 = Color(0xFFFEDBD0);
const Color kShrinePink300 = Color(0xFFFBB8AC);
const Color kShrinePink400 = Color(0xFFEAA4A4);
const Color kShrineBrown900 = Color(0xFF442B2D);
const Color kShrineBrown600 = Color(0xFF7D4F52);
const Color kShrineErrorRed = Color(0xFFC5032B);
const Color kShrineSurfaceWhite = Color(0xFFFFFBFA);
const Color kShrineBackgroundWhite = Colors.white;
| flutter/dev/integration_tests/flutter_gallery/lib/demo/shrine/colors.dart/0 | {
"file_path": "flutter/dev/integration_tests/flutter_gallery/lib/demo/shrine/colors.dart",
"repo_id": "flutter",
"token_count": 225
} | 518 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'transformations_demo_board.dart';
import 'transformations_demo_color_picker.dart';
// The panel for editing a board point.
@immutable
class EditBoardPoint extends StatelessWidget {
const EditBoardPoint({
super.key,
required this.boardPoint,
this.onColorSelection,
});
final BoardPoint boardPoint;
final ValueChanged<Color>? onColorSelection;
@override
Widget build (BuildContext context) {
return Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Text(
'${boardPoint.q}, ${boardPoint.r}',
textAlign: TextAlign.right,
style: const TextStyle(fontWeight: FontWeight.bold),
),
ColorPicker(
colors: boardPointColors,
selectedColor: boardPoint.color,
onColorSelection: onColorSelection,
),
],
);
}
}
| flutter/dev/integration_tests/flutter_gallery/lib/demo/transformations/transformations_demo_edit_board_point.dart/0 | {
"file_path": "flutter/dev/integration_tests/flutter_gallery/lib/demo/transformations/transformations_demo_edit_board_point.dart",
"repo_id": "flutter",
"token_count": 407
} | 519 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
@immutable
class GalleryTextScaleValue {
const GalleryTextScaleValue(this.scale, this.label);
final double? scale;
final String label;
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is GalleryTextScaleValue
&& other.scale == scale
&& other.label == label;
}
@override
int get hashCode => Object.hash(scale, label);
@override
String toString() {
return '$runtimeType($label)';
}
}
const List<GalleryTextScaleValue> kAllGalleryTextScaleValues = <GalleryTextScaleValue>[
GalleryTextScaleValue(null, 'System Default'),
GalleryTextScaleValue(0.8, 'Small'),
GalleryTextScaleValue(1.0, 'Normal'),
GalleryTextScaleValue(1.3, 'Large'),
GalleryTextScaleValue(2.0, 'Huge'),
];
@immutable
class GalleryVisualDensityValue {
const GalleryVisualDensityValue(this.visualDensity, this.label);
final VisualDensity visualDensity;
final String label;
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is GalleryVisualDensityValue
&& other.visualDensity == visualDensity
&& other.label == label;
}
@override
int get hashCode => Object.hash(visualDensity, label);
@override
String toString() {
return '$runtimeType($label)';
}
}
const List<GalleryVisualDensityValue> kAllGalleryVisualDensityValues = <GalleryVisualDensityValue>[
GalleryVisualDensityValue(VisualDensity.standard, 'System Default'),
GalleryVisualDensityValue(VisualDensity.comfortable, 'Comfortable'),
GalleryVisualDensityValue(VisualDensity.compact, 'Compact'),
GalleryVisualDensityValue(VisualDensity(horizontal: -3, vertical: -3), 'Very Compact'),
];
| flutter/dev/integration_tests/flutter_gallery/lib/gallery/scales.dart/0 | {
"file_path": "flutter/dev/integration_tests/flutter_gallery/lib/gallery/scales.dart",
"repo_id": "flutter",
"token_count": 633
} | 520 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_gallery/demo/material/drawer_demo.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('Drawer header does not scroll', (WidgetTester tester) async {
await tester.pumpWidget(MaterialApp(
theme: ThemeData(platform: TargetPlatform.iOS),
home: const DrawerDemo(),
));
await tester.tap(find.text('Tap here to open the drawer'));
await tester.pump();
await tester.pump(const Duration(milliseconds: 500));
expect(tester.getTopLeft(find.byType(UserAccountsDrawerHeader)).dy, 0.0);
final double initialTopItemSaneY = tester.getTopLeft(find.text('Drawer item A')).dy;
expect(initialTopItemSaneY, greaterThan(0.0));
await tester.drag(find.text('Drawer item B'), const Offset(0.0, 400.0));
await tester.pump();
expect(tester.getTopLeft(find.byType(UserAccountsDrawerHeader)).dy, 0.0);
expect(tester.getTopLeft(find.text('Drawer item A')).dy, greaterThan(initialTopItemSaneY));
expect(tester.getTopLeft(find.text('Drawer item A')).dy, lessThanOrEqualTo(initialTopItemSaneY + 400.0));
});
}
| flutter/dev/integration_tests/flutter_gallery/test/demo/material/drawer_demo_test.dart/0 | {
"file_path": "flutter/dev/integration_tests/flutter_gallery/test/demo/material/drawer_demo_test.dart",
"repo_id": "flutter",
"token_count": 474
} | 521 |
name: gradle_deprecated_settings
description: Integration test for the current settings.gradle.
environment:
sdk: '>=3.2.0-0 <4.0.0'
dependencies:
flutter:
sdk: flutter
camera: 0.10.5+9
camera_android: 0.10.8+16 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
camera_avfoundation: 0.9.14+1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
camera_platform_interface: 2.7.4 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
camera_web: 0.3.2+4 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
characters: 1.3.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
collection: 1.18.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
cross_file: 0.3.4+1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
flutter_plugin_android_lifecycle: 2.0.17 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
material_color_utilities: 0.8.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
meta: 1.12.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
plugin_platform_interface: 2.1.8 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
stream_transform: 2.1.0 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
vector_math: 2.1.4 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
web: 0.5.1 # THIS LINE IS AUTOGENERATED - TO UPDATE USE "flutter update-packages --force-upgrade"
flutter:
uses-material-design: true
# PUBSPEC CHECKSUM: 81c3
| flutter/dev/integration_tests/gradle_deprecated_settings/pubspec.yaml/0 | {
"file_path": "flutter/dev/integration_tests/gradle_deprecated_settings/pubspec.yaml",
"repo_id": "flutter",
"token_count": 625
} | 522 |
<?xml version="1.0" encoding="UTF-8"?>
<document type="com.apple.InterfaceBuilder3.CocoaTouch.Storyboard.XIB" version="3.0" toolsVersion="14313.18" targetRuntime="iOS.CocoaTouch" propertyAccessControl="none" useAutolayout="YES" launchScreen="YES" useTraitCollections="YES" useSafeAreas="YES" colorMatched="YES" initialViewController="01J-lp-oVM">
<device id="retina4_7" orientation="portrait">
<adaptation id="fullscreen"/>
</device>
<dependencies>
<plugIn identifier="com.apple.InterfaceBuilder.IBCocoaTouchPlugin" version="14283.14"/>
<capability name="Safe area layout guides" minToolsVersion="9.0"/>
<capability name="documents saved in the Xcode 8 format" minToolsVersion="8.0"/>
</dependencies>
<scenes>
<!--View Controller-->
<scene sceneID="EHf-IW-A2E">
<objects>
<viewController id="01J-lp-oVM" sceneMemberID="viewController">
<view key="view" contentMode="scaleToFill" id="Ze5-6b-2t3">
<rect key="frame" x="0.0" y="0.0" width="375" height="667"/>
<autoresizingMask key="autoresizingMask" widthSizable="YES" heightSizable="YES"/>
<subviews>
<label opaque="NO" clipsSubviews="YES" userInteractionEnabled="NO" contentMode="left" horizontalHuggingPriority="251" verticalHuggingPriority="251" text="© 2018 The Flutter Authors. All rights reserved." textAlignment="center" lineBreakMode="tailTruncation" baselineAdjustment="alignBaselines" minimumFontSize="9" translatesAutoresizingMaskIntoConstraints="NO" id="obG-Y5-kRd">
<rect key="frame" x="0.0" y="626" width="375" height="21"/>
<fontDescription key="fontDescription" type="system" pointSize="17"/>
<color key="textColor" red="0.0" green="0.0" blue="0.0" alpha="1" colorSpace="custom" customColorSpace="sRGB"/>
<nil key="highlightedColor"/>
</label>
<label opaque="NO" clipsSubviews="YES" userInteractionEnabled="NO" contentMode="left" horizontalHuggingPriority="251" verticalHuggingPriority="251" text="ios_add2app" textAlignment="center" lineBreakMode="middleTruncation" baselineAdjustment="alignBaselines" minimumFontSize="18" translatesAutoresizingMaskIntoConstraints="NO" id="GJd-Yh-RWb">
<rect key="frame" x="0.0" y="202" width="375" height="43"/>
<fontDescription key="fontDescription" type="boldSystem" pointSize="36"/>
<color key="textColor" red="0.0" green="0.0" blue="0.0" alpha="1" colorSpace="custom" customColorSpace="sRGB"/>
<nil key="highlightedColor"/>
</label>
</subviews>
<color key="backgroundColor" red="1" green="1" blue="1" alpha="1" colorSpace="custom" customColorSpace="sRGB"/>
<constraints>
<constraint firstItem="Bcu-3y-fUS" firstAttribute="centerX" secondItem="obG-Y5-kRd" secondAttribute="centerX" id="5cz-MP-9tL"/>
<constraint firstItem="Bcu-3y-fUS" firstAttribute="centerX" secondItem="GJd-Yh-RWb" secondAttribute="centerX" id="Q3B-4B-g5h"/>
<constraint firstItem="obG-Y5-kRd" firstAttribute="leading" secondItem="Bcu-3y-fUS" secondAttribute="leading" symbolic="YES" id="SfN-ll-jLj"/>
<constraint firstAttribute="bottom" secondItem="obG-Y5-kRd" secondAttribute="bottom" constant="20" id="Y44-ml-fuU"/>
<constraint firstItem="GJd-Yh-RWb" firstAttribute="centerY" secondItem="Ze5-6b-2t3" secondAttribute="bottom" multiplier="1/3" constant="1" id="moa-c2-u7t"/>
<constraint firstItem="GJd-Yh-RWb" firstAttribute="leading" secondItem="Bcu-3y-fUS" secondAttribute="leading" constant="20" symbolic="YES" id="x7j-FC-K8j"/>
</constraints>
<viewLayoutGuide key="safeArea" id="Bcu-3y-fUS"/>
</view>
</viewController>
<placeholder placeholderIdentifier="IBFirstResponder" id="iYj-Kq-Ea1" userLabel="First Responder" sceneMemberID="firstResponder"/>
</objects>
<point key="canvasLocation" x="53" y="375"/>
</scene>
</scenes>
</document>
| flutter/dev/integration_tests/ios_add2app_life_cycle/ios_add2app/Launch Screen.storyboard/0 | {
"file_path": "flutter/dev/integration_tests/ios_add2app_life_cycle/ios_add2app/Launch Screen.storyboard",
"repo_id": "flutter",
"token_count": 2138
} | 523 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'dart:ui' as ui;
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:ffi_package/ffi_package.dart';
import 'marquee.dart';
/// Route names. (See [main] for more details.)
///
/// The route names must match those sent by the platform-specific component.
const String greenMarqueeRouteName = 'marquee_green';
const String purpleMarqueeRouteName = 'marquee_purple';
const String fullscreenRouteName = 'full';
const String hybridRouteName = 'hybrid';
/// Channel used to let the Flutter app know to reset the app to a specific
/// route. See the [run] method.
///
/// We shouldn't use the `setInitialRoute` method on the system
/// navigation channel, as that never gets propagated back to Flutter
/// after the initial call.
const String _kReloadChannelName = 'reload';
const BasicMessageChannel<String> _kReloadChannel =
BasicMessageChannel<String>(_kReloadChannelName, StringCodec());
void main() {
// Ensures bindings are initialized before doing anything.
WidgetsFlutterBinding.ensureInitialized();
// Start listening immediately for messages from the iOS side. ObjC calls
// will be made to let us know when we should be changing the app state.
_kReloadChannel.setMessageHandler(run);
// Start off with whatever the initial route is supposed to be.
run(ui.window.defaultRouteName);
}
Future<String> run(String? name) async {
// The platform-specific component will call [setInitialRoute] on the Flutter
// view (or view controller for iOS) to set [ui.window.defaultRouteName].
// We then dispatch based on the route names to show different Flutter
// widgets.
// Since we don't really care about Flutter-side navigation in this app, we're
// not using a regular routes map.
name ??= '';
switch (name) {
case greenMarqueeRouteName:
runApp(Marquee(color: Colors.green[400]));
break;
case purpleMarqueeRouteName:
runApp(Marquee(color: Colors.purple[400]));
break;
case fullscreenRouteName:
case hybridRouteName:
default:
runApp(FlutterView(initialRoute: name));
break;
}
return '';
}
class FlutterView extends StatelessWidget {
const FlutterView({required this.initialRoute});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter View',
home: MyHomePage(initialRoute: initialRoute),
);
}
final String initialRoute;
}
class MyHomePage extends StatefulWidget {
const MyHomePage({required this.initialRoute});
@override
_MyHomePageState createState() => _MyHomePageState();
final String initialRoute;
/// Whether we should display the home page in fullscreen mode.
///
/// If in full screen mode, we will use an [AppBar] widget to show our own
/// title.
bool get isFullscreen => initialRoute == fullscreenRouteName;
/// Whether tapping the Flutter button should notify an external source.
///
/// If false, the button will increments our own internal counter.
bool get hasExternalTarget => initialRoute == hybridRouteName;
}
class _MyHomePageState extends State<MyHomePage> {
// The name of the message channel used to communicate with the
// platform-specific component.
//
// This string must match the one used on the platform side.
static const String _channel = 'increment';
// The message to send to the platform-specific component when our button
// is tapped.
static const String _pong = 'pong';
// Used to pass messages between the platform-specific component and the
// Flutter component.
static const BasicMessageChannel<String> _platform =
BasicMessageChannel<String>(_channel, StringCodec());
// An internal count. Normally this represents the number of times that the
// button on the Flutter page has been tapped.
int _counter = 0;
late int sumResult;
late Future<int> sumAsyncResult;
@override
void initState() {
super.initState();
_platform.setMessageHandler(_handlePlatformIncrement);
sumResult = sum(1, 2);
sumAsyncResult = sumAsync(3, 4);
}
/// Directly increments our internal counter and rebuilds the UI.
void _incrementCounter() {
setState(() {
_counter++;
});
}
/// Callback for messages sent by the platform-specific component.
///
/// Increments our internal counter.
Future<String> _handlePlatformIncrement(String? message) async {
// Normally we'd dispatch based on the value of [message], but in this
// sample, there is only one message that is sent to us.
_incrementCounter();
return '';
}
/// Sends a message to the platform-specific component to increment its
/// counter.
void _sendFlutterIncrement() {
_platform.send(_pong);
}
@override
Widget build(BuildContext context) {
final String buttonName =
widget.hasExternalTarget ? 'Platform button' : 'Button';
return Scaffold(
appBar: widget.isFullscreen
? AppBar(title: const Text('Fullscreen Flutter'))
: null,
body: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Expanded(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Center(
child: Text(
'$buttonName tapped $_counter time${_counter == 1 ? '' : 's'}.',
style: const TextStyle(fontSize: 17.0),
),
),
const TextButton(
child: Text('POP'),
onPressed: SystemNavigator.pop,
),
],
),
),
Container(
padding: const EdgeInsets.only(bottom: 15.0, left: 5.0),
child: Row(
children: const <Widget>[
Text('Flutter', style: TextStyle(fontSize: 30.0)),
],
),
),
],
),
floatingActionButton: Semantics(
label: 'Increment via Flutter',
child: FloatingActionButton(
onPressed: widget.hasExternalTarget
? _sendFlutterIncrement
: _incrementCounter,
child: const Icon(Icons.add),
),
),
);
}
}
| flutter/dev/integration_tests/ios_host_app/flutterapp/lib/main/0 | {
"file_path": "flutter/dev/integration_tests/ios_host_app/flutterapp/lib/main",
"repo_id": "flutter",
"token_count": 2273
} | 524 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:dual_screen/dual_screen.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'deferred_widget.dart';
import 'main.dart';
import 'pages/demo.dart';
import 'pages/home.dart';
import 'studies/crane/app.dart' deferred as crane;
import 'studies/crane/routes.dart' as crane_routes;
import 'studies/fortnightly/app.dart' deferred as fortnightly;
import 'studies/fortnightly/routes.dart' as fortnightly_routes;
import 'studies/rally/app.dart' deferred as rally;
import 'studies/rally/routes.dart' as rally_routes;
import 'studies/reply/app.dart' as reply;
import 'studies/reply/routes.dart' as reply_routes;
import 'studies/shrine/app.dart' deferred as shrine;
import 'studies/shrine/routes.dart' as shrine_routes;
import 'studies/starter/app.dart' as starter_app;
import 'studies/starter/routes.dart' as starter_app_routes;
typedef PathWidgetBuilder = Widget Function(BuildContext, String?);
class Path {
const Path(this.pattern, this.builder, {this.openInSecondScreen = false});
/// A RegEx string for route matching.
final String pattern;
/// The builder for the associated pattern route. The first argument is the
/// [BuildContext] and the second argument a RegEx match if that is included
/// in the pattern.
///
/// ```dart
/// Path(
/// 'r'^/demo/([\w-]+)$',
/// (context, matches) => Page(argument: match),
/// )
/// ```
final PathWidgetBuilder builder;
/// If the route should open on the second screen on foldables.
final bool openInSecondScreen;
}
class RouteConfiguration {
/// List of [Path] to for route matching. When a named route is pushed with
/// [Navigator.pushNamed], the route name is matched with the [Path.pattern]
/// in the list below. As soon as there is a match, the associated builder
/// will be returned. This means that the paths higher up in the list will
/// take priority.
static List<Path> paths = <Path>[
Path(
r'^' + DemoPage.baseRoute + r'/([\w-]+)$',
(BuildContext context, String? match) => DemoPage(slug: match),
),
Path(
r'^' + rally_routes.homeRoute,
(BuildContext context, String? match) => StudyWrapper(
study: DeferredWidget(rally.loadLibrary,
() => rally.RallyApp()), // ignore: prefer_const_constructors
),
openInSecondScreen: true,
),
Path(
r'^' + shrine_routes.homeRoute,
(BuildContext context, String? match) => StudyWrapper(
study: DeferredWidget(shrine.loadLibrary,
() => shrine.ShrineApp()), // ignore: prefer_const_constructors
),
openInSecondScreen: true,
),
Path(
r'^' + crane_routes.defaultRoute,
(BuildContext context, String? match) => StudyWrapper(
study: DeferredWidget(crane.loadLibrary,
() => crane.CraneApp(), // ignore: prefer_const_constructors
placeholder: const DeferredLoadingPlaceholder(name: 'Crane')),
),
openInSecondScreen: true,
),
Path(
r'^' + fortnightly_routes.defaultRoute,
(BuildContext context, String? match) => StudyWrapper(
study: DeferredWidget(
fortnightly.loadLibrary,
// ignore: prefer_const_constructors
() => fortnightly.FortnightlyApp()),
),
openInSecondScreen: true,
),
Path(
r'^' + reply_routes.homeRoute,
// ignore: prefer_const_constructors
(BuildContext context, String? match) =>
const StudyWrapper(study: reply.ReplyApp(), hasBottomNavBar: true),
openInSecondScreen: true,
),
Path(
r'^' + starter_app_routes.defaultRoute,
(BuildContext context, String? match) => const StudyWrapper(
study: starter_app.StarterApp(),
),
openInSecondScreen: true,
),
Path(
r'^/',
(BuildContext context, String? match) => const RootPage(),
),
];
/// The route generator callback used when the app is navigated to a named
/// route. Set it on the [MaterialApp.onGenerateRoute] or
/// [WidgetsApp.onGenerateRoute] to make use of the [paths] for route
/// matching.
static Route<dynamic>? onGenerateRoute(
RouteSettings settings,
bool hasHinge,
) {
for (final Path path in paths) {
final RegExp regExpPattern = RegExp(path.pattern);
if (regExpPattern.hasMatch(settings.name!)) {
final RegExpMatch firstMatch = regExpPattern.firstMatch(settings.name!)!;
final String? match = (firstMatch.groupCount == 1) ? firstMatch.group(1) : null;
if (kIsWeb) {
return NoAnimationMaterialPageRoute<void>(
builder: (BuildContext context) => path.builder(context, match),
settings: settings,
);
}
if (path.openInSecondScreen && hasHinge) {
return TwoPanePageRoute<void>(
builder: (BuildContext context) => path.builder(context, match),
settings: settings,
);
} else {
return MaterialPageRoute<void>(
builder: (BuildContext context) => path.builder(context, match),
settings: settings,
);
}
}
}
// If no match was found, we let [WidgetsApp.onUnknownRoute] handle it.
return null;
}
}
class NoAnimationMaterialPageRoute<T> extends MaterialPageRoute<T> {
NoAnimationMaterialPageRoute({
required super.builder,
super.settings,
});
@override
Widget buildTransitions(
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
Widget child,
) {
return child;
}
}
class TwoPanePageRoute<T> extends OverlayRoute<T> {
TwoPanePageRoute({
required this.builder,
super.settings,
});
final WidgetBuilder builder;
@override
Iterable<OverlayEntry> createOverlayEntries() sync* {
yield OverlayEntry(builder: (BuildContext context) {
final Rect? hinge = MediaQuery.of(context).hinge?.bounds;
if (hinge == null) {
return builder.call(context);
} else {
return Positioned(
top: 0,
left: hinge.right,
right: 0,
bottom: 0,
child: builder.call(context));
}
});
}
}
| flutter/dev/integration_tests/new_gallery/lib/routes.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/routes.dart",
"repo_id": "flutter",
"token_count": 2460
} | 525 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import '../../data/gallery_options.dart';
import '../../gallery_localizations.dart';
import '../../layout/adaptive.dart';
import '../../layout/image_placeholder.dart';
import '../../layout/text_scale.dart';
import 'routes.dart' as routes;
import 'shared.dart';
const String _fortnightlyTitle = 'Fortnightly';
class FortnightlyApp extends StatelessWidget {
const FortnightlyApp({super.key});
static const String defaultRoute = routes.defaultRoute;
@override
Widget build(BuildContext context) {
final StatelessWidget home = isDisplayDesktop(context)
? const _FortnightlyHomeDesktop()
: const _FortnightlyHomeMobile();
return MaterialApp(
restorationScopeId: 'fortnightly_app',
title: _fortnightlyTitle,
debugShowCheckedModeBanner: false,
theme: buildTheme(context).copyWith(
platform: GalleryOptions.of(context).platform,
),
home: ApplyTextOptions(child: home),
routes: <String, WidgetBuilder>{
FortnightlyApp.defaultRoute: (BuildContext context) => ApplyTextOptions(child: home),
},
initialRoute: FortnightlyApp.defaultRoute,
// L10n settings.
localizationsDelegates: GalleryLocalizations.localizationsDelegates,
supportedLocales: GalleryLocalizations.supportedLocales,
locale: GalleryOptions.of(context).locale,
);
}
}
class _FortnightlyHomeMobile extends StatelessWidget {
const _FortnightlyHomeMobile();
@override
Widget build(BuildContext context) {
return Scaffold(
drawer: const Drawer(
child: SafeArea(
child: NavigationMenu(isCloseable: true),
),
),
appBar: AppBar(
automaticallyImplyLeading: false,
title: Semantics(
label: _fortnightlyTitle,
child: const FadeInImagePlaceholder(
image: AssetImage(
'fortnightly/fortnightly_title.png',
package: 'flutter_gallery_assets',
),
placeholder: SizedBox.shrink(),
excludeFromSemantics: true,
),
),
actions: <Widget>[
IconButton(
icon: const Icon(Icons.search),
tooltip: GalleryLocalizations.of(context)!.shrineTooltipSearch,
onPressed: () {},
),
],
),
body: SafeArea(
child: ListView(
restorationId: 'list_view',
children: <Widget>[
const HashtagBar(),
for (final Widget item in buildArticlePreviewItems(context))
Padding(
padding: const EdgeInsets.symmetric(horizontal: 16),
child: item,
),
],
),
),
);
}
}
class _FortnightlyHomeDesktop extends StatelessWidget {
const _FortnightlyHomeDesktop();
@override
Widget build(BuildContext context) {
const double menuWidth = 200.0;
const SizedBox spacer = SizedBox(width: 20);
final double headerHeight = 40 * reducedTextScale(context);
return Scaffold(
body: Padding(
padding: const EdgeInsets.all(16),
child: Column(
children: <Widget>[
SizedBox(
height: headerHeight,
child: Row(
children: <Widget>[
Container(
width: menuWidth,
alignment: AlignmentDirectional.centerStart,
margin: const EdgeInsets.only(left: 12),
child: Semantics(
label: _fortnightlyTitle,
child: Image.asset(
'fortnightly/fortnightly_title.png',
package: 'flutter_gallery_assets',
excludeFromSemantics: true,
),
),
),
spacer,
const Flexible(
flex: 2,
child: HashtagBar(),
),
spacer,
Flexible(
fit: FlexFit.tight,
child: Container(
alignment: AlignmentDirectional.centerEnd,
child: IconButton(
icon: const Icon(Icons.search),
tooltip: GalleryLocalizations.of(context)!
.shrineTooltipSearch,
onPressed: () {},
),
),
),
],
),
),
Flexible(
child: Row(
children: <Widget>[
const SizedBox(
width: menuWidth,
child: NavigationMenu(),
),
spacer,
Flexible(
flex: 2,
child: ListView(
children: buildArticlePreviewItems(context),
),
),
spacer,
Flexible(
fit: FlexFit.tight,
child: ListView(
children: <Widget>[
...buildStockItems(context),
const SizedBox(height: 32),
...buildVideoPreviewItems(context),
],
),
),
],
),
),
],
),
),
);
}
}
| flutter/dev/integration_tests/new_gallery/lib/studies/fortnightly/app.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/studies/fortnightly/app.dart",
"repo_id": "flutter",
"token_count": 2964
} | 526 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import '../../../gallery_localizations.dart';
import '../charts/pie_chart.dart';
import '../data.dart';
import '../finance.dart';
import 'sidebar.dart';
class BudgetsView extends StatefulWidget {
const BudgetsView({super.key});
@override
State<BudgetsView> createState() => _BudgetsViewState();
}
class _BudgetsViewState extends State<BudgetsView>
with SingleTickerProviderStateMixin {
@override
Widget build(BuildContext context) {
final List<BudgetData> items = DummyDataService.getBudgetDataList(context);
final double capTotal = sumBudgetDataPrimaryAmount(items);
final double usedTotal = sumBudgetDataAmountUsed(items);
final List<UserDetailData> detailItems = DummyDataService.getBudgetDetailList(
context,
capTotal: capTotal,
usedTotal: usedTotal,
);
return TabWithSidebar(
restorationId: 'budgets_view',
mainView: FinancialEntityView(
heroLabel: GalleryLocalizations.of(context)!.rallyBudgetLeft,
heroAmount: capTotal - usedTotal,
segments: buildSegmentsFromBudgetItems(items),
wholeAmount: capTotal,
financialEntityCards: buildBudgetDataListViews(items, context),
),
sidebarItems: <Widget>[
for (final UserDetailData item in detailItems)
SidebarItem(title: item.title, value: item.value)
],
);
}
}
| flutter/dev/integration_tests/new_gallery/lib/studies/rally/tabs/budgets.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/studies/rally/tabs/budgets.dart",
"repo_id": "flutter",
"token_count": 557
} | 527 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:provider/provider.dart';
import 'model/email_store.dart';
class SearchPage extends StatelessWidget {
const SearchPage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
body: SafeArea(
child: Material(
color: Theme.of(context).colorScheme.surface,
child: Column(
children: <Widget>[
Padding(
padding: const EdgeInsets.all(8),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
BackButton(
key: const ValueKey<String>('ReplyExit'),
onPressed: () {
Provider.of<EmailStore>(
context,
listen: false,
).onSearchPage = false;
},
),
const Expanded(
child: TextField(
decoration: InputDecoration.collapsed(
hintText: 'Search email',
),
),
),
IconButton(
icon: const Icon(Icons.mic),
onPressed: () {},
)
],
),
),
const Divider(thickness: 1),
const Expanded(
child: SingleChildScrollView(
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
_SectionHeader(title: 'YESTERDAY'),
_SearchHistoryTile(
search: '481 Van Brunt Street',
address: 'Brooklyn, NY',
),
_SearchHistoryTile(
icon: Icons.home,
search: 'Home',
address: '199 Pacific Street, Brooklyn, NY',
),
_SectionHeader(title: 'THIS WEEK'),
_SearchHistoryTile(
search: 'BEP GA',
address: 'Forsyth Street, New York, NY',
),
_SearchHistoryTile(
search: 'Sushi Nakazawa',
address: 'Commerce Street, New York, NY',
),
_SearchHistoryTile(
search: 'IFC Center',
address: '6th Avenue, New York, NY',
),
],
),
),
),
],
),
),
),
);
}
}
class _SectionHeader extends StatelessWidget {
const _SectionHeader({required this.title});
final String title;
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsetsDirectional.only(
start: 16,
top: 16,
bottom: 16,
),
child: Text(
title,
style: Theme.of(context).textTheme.labelLarge,
),
);
}
}
class _SearchHistoryTile extends StatelessWidget {
const _SearchHistoryTile({
this.icon = Icons.access_time,
required this.search,
required this.address,
});
final IconData icon;
final String search;
final String address;
@override
Widget build(BuildContext context) {
return ListTile(
leading: Icon(icon),
title: Text(search),
subtitle: Text(address),
onTap: () {},
);
}
}
| flutter/dev/integration_tests/new_gallery/lib/studies/reply/search_page.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/studies/reply/search_page.dart",
"repo_id": "flutter",
"token_count": 2192
} | 528 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:math';
import 'package:flutter/material.dart';
import '../../../data/gallery_options.dart';
import '../../../layout/text_scale.dart';
import '../category_menu_page.dart';
import '../model/product.dart';
import '../page_status.dart';
import 'balanced_layout.dart';
import 'desktop_product_columns.dart';
import 'product_card.dart';
import 'product_columns.dart';
const double _topPadding = 34.0;
const double _bottomPadding = 44.0;
const double _cardToScreenWidthRatio = 0.59;
class MobileAsymmetricView extends StatelessWidget {
const MobileAsymmetricView({
super.key,
required this.products,
});
final List<Product> products;
List<SizedBox> _buildColumns(
BuildContext context,
BoxConstraints constraints,
) {
if (products.isEmpty) {
return const <SizedBox>[];
}
// Decide whether the page size and text size allow 2-column products.
final double cardHeight = (constraints.biggest.height -
_topPadding -
_bottomPadding -
TwoProductCardColumn.spacerHeight) /
2;
final double imageWidth = _cardToScreenWidthRatio * constraints.biggest.width -
TwoProductCardColumn.horizontalPadding;
final double imageHeight = cardHeight -
MobileProductCard.defaultTextBoxHeight *
GalleryOptions.of(context).textScaleFactor(context);
final bool shouldUseAlternatingLayout =
imageHeight > 0 && imageWidth / imageHeight < 49 / 33;
if (shouldUseAlternatingLayout) {
// Alternating layout: a layout of alternating 2-product
// and 1-product columns.
//
// This will return a list of columns. It will oscillate between the two
// kinds of columns. Even cases of the index (0, 2, 4, etc) will be
// TwoProductCardColumn and the odd cases will be OneProductCardColumn.
//
// Each pair of columns will advance us 3 products forward (2 + 1). That's
// some kinda awkward math so we use _evenCasesIndex and _oddCasesIndex as
// helpers for creating the index of the product list that will correspond
// to the index of the list of columns.
return List<SizedBox>.generate(_listItemCount(products.length), (int index) {
double width = _cardToScreenWidthRatio * MediaQuery.of(context).size.width;
Widget column;
if (index.isEven) {
/// Even cases
final int bottom = _evenCasesIndex(index);
column = TwoProductCardColumn(
bottom: products[bottom],
top:
products.length - 1 >= bottom + 1 ? products[bottom + 1] : null,
imageAspectRatio: imageWidth / imageHeight,
);
width += 32;
} else {
/// Odd cases
column = OneProductCardColumn(
product: products[_oddCasesIndex(index)],
reverse: true,
);
}
return SizedBox(
width: width,
child: Padding(
padding: const EdgeInsets.symmetric(horizontal: 16),
child: column,
),
);
}).toList();
} else {
// Alternating layout: a layout of 1-product columns.
return <SizedBox>[
for (final Product product in products)
SizedBox(
width: _cardToScreenWidthRatio * MediaQuery.of(context).size.width,
child: Padding(
padding: const EdgeInsets.symmetric(horizontal: 16),
child: OneProductCardColumn(
product: product,
reverse: false,
),
),
)
];
}
}
int _evenCasesIndex(int input) {
// The operator ~/ is a cool one. It's the truncating division operator. It
// divides the number and if there's a remainder / decimal, it cuts it off.
// This is like dividing and then casting the result to int. Also, it's
// functionally equivalent to floor() in this case.
return input ~/ 2 * 3;
}
int _oddCasesIndex(int input) {
assert(input > 0);
return (input / 2).ceil() * 3 - 1;
}
int _listItemCount(int totalItems) {
return (totalItems % 3 == 0)
? totalItems ~/ 3 * 2
: (totalItems / 3).ceil() * 2 - 1;
}
@override
Widget build(BuildContext context) {
return AnimatedBuilder(
animation: PageStatus.of(context)!.cartController,
builder: (BuildContext context, Widget? child) => AnimatedBuilder(
animation: PageStatus.of(context)!.menuController,
builder: (BuildContext context, Widget? child) => ExcludeSemantics(
excluding: !productPageIsVisible(context),
child: LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
return ListView(
restorationId: 'product_page_list_view',
scrollDirection: Axis.horizontal,
padding: const EdgeInsetsDirectional.fromSTEB(
0,
_topPadding,
16,
_bottomPadding,
),
physics: const BouncingScrollPhysics(),
children: _buildColumns(context, constraints),
);
},
),
),
),
);
}
}
class DesktopAsymmetricView extends StatelessWidget {
const DesktopAsymmetricView({
super.key,
required this.products,
});
final List<Product> products;
@override
Widget build(BuildContext context) {
// Determine the scale factor for the desktop asymmetric view.
final double textScaleFactor = GalleryOptions.of(context).textScaleFactor(context);
// When text is larger, the images becomes wider, but at half the rate.
final double imageScaleFactor = reducedTextScale(context);
// When text is larger, horizontal padding becomes smaller.
final num paddingScaleFactor = textScaleFactor >= 1.5 ? 0.25 : 1;
// Calculate number of columns
final double sidebar = desktopCategoryMenuPageWidth(context: context);
final num minimumBoundaryWidth = 84 * paddingScaleFactor;
final double columnWidth = 186 * imageScaleFactor;
final double columnGapWidth = 24 * imageScaleFactor;
final double windowWidth = MediaQuery.of(context).size.width;
final int idealColumnCount = max(
1,
((windowWidth + columnGapWidth - 2 * minimumBoundaryWidth - sidebar) /
(columnWidth + columnGapWidth))
.floor(),
);
// Limit column width to fit within window when there is only one column.
final double actualColumnWidth = idealColumnCount == 1
? min(
columnWidth,
windowWidth - sidebar - 2 * minimumBoundaryWidth,
)
: columnWidth;
final int columnCount = min(idealColumnCount, max(products.length, 1));
return AnimatedBuilder(
animation: PageStatus.of(context)!.cartController,
builder: (BuildContext context, Widget? child) => ExcludeSemantics(
excluding: !productPageIsVisible(context),
child: DesktopColumns(
columnCount: columnCount,
products: products,
largeImageWidth: actualColumnWidth,
smallImageWidth: columnCount > 1
? columnWidth - columnGapWidth
: actualColumnWidth,
),
),
);
}
}
class DesktopColumns extends StatelessWidget {
const DesktopColumns({
super.key,
required this.columnCount,
required this.products,
required this.largeImageWidth,
required this.smallImageWidth,
});
final int columnCount;
final List<Product> products;
final double largeImageWidth;
final double smallImageWidth;
@override
Widget build(BuildContext context) {
final Widget gap = Container(width: 24);
final List<List<Product>> productCardLists = balancedLayout(
context: context,
columnCount: columnCount,
products: products,
largeImageWidth: largeImageWidth,
smallImageWidth: smallImageWidth,
);
final List<DesktopProductCardColumn> productCardColumns = List<DesktopProductCardColumn>.generate(
columnCount,
(int column) {
final bool alignToEnd = (column.isOdd) || (column == columnCount - 1);
final bool startLarge = column.isOdd;
final bool lowerStart = column.isOdd;
return DesktopProductCardColumn(
alignToEnd: alignToEnd,
startLarge: startLarge,
lowerStart: lowerStart,
products: productCardLists[column],
largeImageWidth: largeImageWidth,
smallImageWidth: smallImageWidth,
);
},
);
return ListView(
physics: const AlwaysScrollableScrollPhysics(),
children: <Widget>[
Container(height: 60),
Row(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
const Spacer(),
...List<Widget>.generate(
2 * columnCount - 1,
(int generalizedColumnIndex) {
if (generalizedColumnIndex.isEven) {
return productCardColumns[generalizedColumnIndex ~/ 2];
} else {
return gap;
}
},
),
const Spacer(),
],
),
Container(height: 60),
],
);
}
}
| flutter/dev/integration_tests/new_gallery/lib/studies/shrine/supplemental/asymmetric_view.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/lib/studies/shrine/supplemental/asymmetric_view.dart",
"repo_id": "flutter",
"token_count": 3802
} | 529 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:gallery/main.dart';
void main() {
testWidgets('Home page hides settings semantics when closed', (WidgetTester tester) async {
await tester.pumpWidget(const GalleryApp());
await tester.pump(const Duration(seconds: 1));
expect(find.bySemanticsLabel('Settings'), findsOneWidget);
expect(find.bySemanticsLabel('Close settings'), findsNothing);
await tester.tap(find.bySemanticsLabel('Settings'));
await tester.pump(const Duration(seconds: 1));
// The test no longer finds Setting and Close settings since the semantics
// are excluded when settings mode is activated.
expect(find.bySemanticsLabel('Settings'), findsNothing);
expect(find.bySemanticsLabel('Close settings'), findsOneWidget);
});
testWidgets('Home page list view is the primary list view', (WidgetTester tester) async {
await tester.pumpWidget(const GalleryApp());
await tester.pumpAndSettle();
final ListView listview =
tester.widget(find.byKey(const ValueKey<String>('HomeListView')));
expect(listview.primary, true);
});
}
| flutter/dev/integration_tests/new_gallery/test/pages/home_test.dart/0 | {
"file_path": "flutter/dev/integration_tests/new_gallery/test/pages/home_test.dart",
"repo_id": "flutter",
"token_count": 411
} | 530 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
const String kDefaultTextField = 'default_textfield';
const String kHeightText = 'height_text';
const String kUnfocusButton = 'unfocus_button';
const String kOffsetText = 'offset_text';
const String kListView = 'list_view';
const String kKeyboardVisibleView = 'keyboard_visible';
| flutter/dev/integration_tests/ui/lib/keys.dart/0 | {
"file_path": "flutter/dev/integration_tests/ui/lib/keys.dart",
"repo_id": "flutter",
"token_count": 122
} | 531 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_driver/flutter_driver.dart';
import 'package:test/test.dart' hide TypeMatcher, isInstanceOf;
// Connect and disconnect from the empty app.
void main() {
group('FlutterDriver', () {
late FlutterDriver driver;
setUpAll(() async {
driver = await FlutterDriver.connect();
});
tearDownAll(() async {
await driver.close();
});
test('empty', () async {}, timeout: Timeout.none);
});
}
| flutter/dev/integration_tests/ui/test_driver/empty_test.dart/0 | {
"file_path": "flutter/dev/integration_tests/ui/test_driver/empty_test.dart",
"repo_id": "flutter",
"token_count": 199
} | 532 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// @dart = 2.12
import 'dart:html' as html;
// Verify that web applications can be run in sound mode.
void main() {
const bool isWeak = <int?>[] is List<int>;
String output;
if (isWeak) {
output = '--- TEST FAILED ---';
} else {
output = '--- TEST SUCCEEDED ---';
}
print(output);
html.HttpRequest.request(
'/test-result',
method: 'POST',
sendData: output,
);
}
| flutter/dev/integration_tests/web/lib/sound_mode.dart/0 | {
"file_path": "flutter/dev/integration_tests/web/lib/sound_mode.dart",
"repo_id": "flutter",
"token_count": 197
} | 533 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
void main() {
runApp(const MaterialApp(
title: 'Focus Demo',
home: FocusDemo(),
));
}
class DemoButton extends StatefulWidget {
const DemoButton({super.key, required this.name, this.canRequestFocus = true, this.autofocus = false});
final String name;
final bool canRequestFocus;
final bool autofocus;
@override
State<DemoButton> createState() => _DemoButtonState();
}
class _DemoButtonState extends State<DemoButton> {
late final FocusNode focusNode = FocusNode(
debugLabel: widget.name,
canRequestFocus: widget.canRequestFocus,
);
@override
void dispose() {
focusNode.dispose();
super.dispose();
}
@override
void didUpdateWidget(DemoButton oldWidget) {
super.didUpdateWidget(oldWidget);
focusNode.canRequestFocus = widget.canRequestFocus;
}
void _handleOnPressed() {
focusNode.requestFocus();
print('Button ${widget.name} pressed.');
debugDumpFocusTree();
}
@override
Widget build(BuildContext context) {
return TextButton(
focusNode: focusNode,
autofocus: widget.autofocus,
style: ButtonStyle(
overlayColor: MaterialStateProperty.resolveWith<Color>((Set<MaterialState> states) {
if (states.contains(MaterialState.focused)) {
return Colors.red.withOpacity(0.25);
}
if (states.contains(MaterialState.hovered)) {
return Colors.blue.withOpacity(0.25);
}
return Colors.transparent;
}),
),
onPressed: () => _handleOnPressed(),
child: Text(widget.name),
);
}
}
class FocusDemo extends StatefulWidget {
const FocusDemo({super.key});
@override
State<FocusDemo> createState() => _FocusDemoState();
}
class _FocusDemoState extends State<FocusDemo> {
FocusNode? outlineFocus;
@override
void initState() {
super.initState();
outlineFocus = FocusNode(debugLabel: 'Demo Focus Node');
}
@override
void dispose() {
outlineFocus?.dispose();
super.dispose();
}
KeyEventResult _handleKeyPress(FocusNode node, KeyEvent event) {
if (event is KeyDownEvent) {
print('Scope got key event: ${event.logicalKey}, $node');
print('Keys down: ${HardwareKeyboard.instance.logicalKeysPressed}');
if (event.logicalKey == LogicalKeyboardKey.tab) {
debugDumpFocusTree();
if (HardwareKeyboard.instance.logicalKeysPressed.contains(LogicalKeyboardKey.shiftLeft)
|| HardwareKeyboard.instance.logicalKeysPressed.contains(LogicalKeyboardKey.shiftRight)) {
print('Moving to previous.');
node.previousFocus();
return KeyEventResult.handled;
} else {
print('Moving to next.');
node.nextFocus();
return KeyEventResult.handled;
}
}
if (event.logicalKey == LogicalKeyboardKey.arrowLeft) {
node.focusInDirection(TraversalDirection.left);
return KeyEventResult.handled;
}
if (event.logicalKey == LogicalKeyboardKey.arrowRight) {
node.focusInDirection(TraversalDirection.right);
return KeyEventResult.handled;
}
if (event.logicalKey == LogicalKeyboardKey.arrowUp) {
node.focusInDirection(TraversalDirection.up);
return KeyEventResult.handled;
}
if (event.logicalKey == LogicalKeyboardKey.arrowDown) {
node.focusInDirection(TraversalDirection.down);
return KeyEventResult.handled;
}
}
return KeyEventResult.ignored;
}
@override
Widget build(BuildContext context) {
final TextTheme textTheme = Theme.of(context).textTheme;
return FocusTraversalGroup(
policy: ReadingOrderTraversalPolicy(),
child: FocusScope(
debugLabel: 'Scope',
onKeyEvent: _handleKeyPress,
autofocus: true,
child: DefaultTextStyle(
style: textTheme.headlineMedium!,
child: Scaffold(
appBar: AppBar(
title: const Text('Focus Demo'),
),
floatingActionButton: FloatingActionButton(
child: const Text('+'),
onPressed: () {},
),
body: Center(
child: Builder(builder: (BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
DemoButton(
name: 'One',
autofocus: true,
),
],
),
const Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
DemoButton(name: 'Two'),
DemoButton(
name: 'Three',
canRequestFocus: false,
),
],
),
const Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
DemoButton(name: 'Four'),
DemoButton(name: 'Five'),
DemoButton(name: 'Six'),
],
),
OutlinedButton(onPressed: () => print('pressed'), child: const Text('PRESS ME')),
const Padding(
padding: EdgeInsets.all(8.0),
child: TextField(
decoration: InputDecoration(labelText: 'Enter Text', filled: true),
),
),
const Padding(
padding: EdgeInsets.all(8.0),
child: TextField(
decoration: InputDecoration(
border: OutlineInputBorder(),
labelText: 'Enter Text',
filled: false,
),
),
),
],
);
}),
),
),
),
),
);
}
}
| flutter/dev/manual_tests/lib/focus.dart/0 | {
"file_path": "flutter/dev/manual_tests/lib/focus.dart",
"repo_id": "flutter",
"token_count": 3188
} | 534 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This script looks at the current commit and branch of the git repository in
// which it was run, and finds the contemporary commit in the master branch of
// another git repository, whose path is provided on the command line. The
// contemporary commit is the one that was public at the time of the last commit
// on the master branch before the current commit's branch was created.
import 'dart:io';
const bool debugLogging = false;
void log(String message) {
if (debugLogging) {
print(message);
}
}
const String _commitTimestampFormat = '--format=%cI';
DateTime _parseTimestamp(String line) => DateTime.parse(line.trim());
int _countLines(String output) => output.trim().split('/n').where((String line) => line.isNotEmpty).length;
String findCommit({
required String primaryRepoDirectory,
required String primaryBranch,
required String primaryTrunk,
required String secondaryRepoDirectory,
required String secondaryBranch,
}) {
final DateTime anchor;
if (primaryBranch == primaryTrunk) {
log('on $primaryTrunk, using last commit time');
anchor = _parseTimestamp(git(primaryRepoDirectory, <String>['log', _commitTimestampFormat, '--max-count=1', primaryBranch, '--']));
} else {
final String mergeBase = git(primaryRepoDirectory, <String>['merge-base', primaryBranch, primaryTrunk], allowFailure: true).trim();
if (mergeBase.isEmpty) {
throw StateError('Branch $primaryBranch does not seem to have a common history with trunk $primaryTrunk.');
}
anchor = _parseTimestamp(git(primaryRepoDirectory, <String>['log', _commitTimestampFormat, '--max-count=1', mergeBase, '--']));
if (debugLogging) {
final int missingTrunkCommits = _countLines(git(primaryRepoDirectory, <String>['rev-list', primaryTrunk, '^$primaryBranch', '--']));
final int extraCommits = _countLines(git(primaryRepoDirectory, <String>['rev-list', primaryBranch, '^$primaryTrunk', '--']));
if (missingTrunkCommits == 0 && extraCommits == 0) {
log('$primaryBranch is even with $primaryTrunk at $mergeBase');
} else {
log('$primaryBranch branched from $primaryTrunk $missingTrunkCommits commits ago, trunk has advanced by $extraCommits commits since then.');
}
}
}
return git(secondaryRepoDirectory, <String>[
'log',
'--format=%H',
'--until=${anchor.toIso8601String()}',
'--max-count=1',
secondaryBranch,
'--',
]);
}
String git(String workingDirectory, List<String> arguments, {bool allowFailure = false}) {
final ProcessResult result = Process.runSync('git', arguments, workingDirectory: workingDirectory);
if (!allowFailure && result.exitCode != 0 || '${result.stderr}'.isNotEmpty) {
throw ProcessException('git', arguments, '${result.stdout}${result.stderr}', result.exitCode);
}
return '${result.stdout}';
}
void main(List<String> arguments) {
if (arguments.isEmpty || arguments.length != 4 || arguments.contains('--help') || arguments.contains('-h')) {
print(
'Usage: dart find_commit.dart <path-to-primary-repo> <primary-trunk> <path-to-secondary-repo> <secondary-branch>\n'
'This script will find the commit in the secondary repo that was contemporary\n'
'when the commit in the primary repo was created. If that commit is on a\n'
"branch, then the date of the branch's last merge is used instead."
);
} else {
final String primaryRepo = arguments.first;
final String primaryTrunk = arguments[1];
final String secondaryRepo = arguments[2];
final String secondaryBranch = arguments.last;
print(findCommit(
primaryRepoDirectory: primaryRepo,
primaryBranch: git(primaryRepo, <String>['rev-parse', '--abbrev-ref', 'HEAD']).trim(),
primaryTrunk: primaryTrunk,
secondaryRepoDirectory: secondaryRepo,
secondaryBranch: secondaryBranch,
).trim());
}
}
| flutter/dev/tools/bin/find_commit.dart/0 | {
"file_path": "flutter/dev/tools/bin/find_commit.dart",
"repo_id": "flutter",
"token_count": 1322
} | 535 |
{
"version": "v0_206",
"md.comp.text-button.container.height": 40.0,
"md.comp.text-button.container.shape": "md.sys.shape.corner.full",
"md.comp.text-button.disabled.label-text.color": "onSurface",
"md.comp.text-button.disabled.label-text.opacity": 0.38,
"md.comp.text-button.focus.indicator.color": "secondary",
"md.comp.text-button.focus.indicator.outline.offset": "md.sys.state.focus-indicator.outer-offset",
"md.comp.text-button.focus.indicator.thickness": "md.sys.state.focus-indicator.thickness",
"md.comp.text-button.focus.label-text.color": "primary",
"md.comp.text-button.focus.state-layer.color": "primary",
"md.comp.text-button.focus.state-layer.opacity": "md.sys.state.focus.state-layer-opacity",
"md.comp.text-button.hover.label-text.color": "primary",
"md.comp.text-button.hover.state-layer.color": "primary",
"md.comp.text-button.hover.state-layer.opacity": "md.sys.state.hover.state-layer-opacity",
"md.comp.text-button.label-text.color": "primary",
"md.comp.text-button.label-text.text-style": "labelLarge",
"md.comp.text-button.pressed.label-text.color": "primary",
"md.comp.text-button.pressed.state-layer.color": "primary",
"md.comp.text-button.pressed.state-layer.opacity": "md.sys.state.pressed.state-layer-opacity",
"md.comp.text-button.with-icon.disabled.icon.color": "onSurface",
"md.comp.text-button.with-icon.disabled.icon.opacity": 0.38,
"md.comp.text-button.with-icon.focus.icon.color": "primary",
"md.comp.text-button.with-icon.hover.icon.color": "primary",
"md.comp.text-button.with-icon.icon.color": "primary",
"md.comp.text-button.with-icon.icon.size": 18.0,
"md.comp.text-button.with-icon.pressed.icon.color": "primary"
}
| flutter/dev/tools/gen_defaults/data/button_text.json/0 | {
"file_path": "flutter/dev/tools/gen_defaults/data/button_text.json",
"repo_id": "flutter",
"token_count": 655
} | 536 |
{
"version": "v0_206",
"md.comp.full-screen-dialog.container.color": "surface",
"md.comp.full-screen-dialog.container.elevation": "md.sys.elevation.level0",
"md.comp.full-screen-dialog.container.shape": "md.sys.shape.corner.none",
"md.comp.full-screen-dialog.header.action.focus.label-text.color": "primary",
"md.comp.full-screen-dialog.header.action.focus.state-layer.color": "primary",
"md.comp.full-screen-dialog.header.action.focus.state-layer.opacity": "md.sys.state.focus.state-layer-opacity",
"md.comp.full-screen-dialog.header.action.hover.label-text.color": "primary",
"md.comp.full-screen-dialog.header.action.hover.state-layer.color": "primary",
"md.comp.full-screen-dialog.header.action.hover.state-layer.opacity": "md.sys.state.hover.state-layer-opacity",
"md.comp.full-screen-dialog.header.action.label-text.color": "primary",
"md.comp.full-screen-dialog.header.action.label-text.text-style": "labelLarge",
"md.comp.full-screen-dialog.header.action.pressed.label-text.color": "primary",
"md.comp.full-screen-dialog.header.action.pressed.state-layer.color": "primary",
"md.comp.full-screen-dialog.header.action.pressed.state-layer.opacity": "md.sys.state.pressed.state-layer-opacity",
"md.comp.full-screen-dialog.header.container.color": "surface",
"md.comp.full-screen-dialog.header.container.elevation": "md.sys.elevation.level0",
"md.comp.full-screen-dialog.header.container.height": 56.0,
"md.comp.full-screen-dialog.header.headline.color": "onSurface",
"md.comp.full-screen-dialog.header.headline.text-style": "titleLarge",
"md.comp.full-screen-dialog.header.icon.color": "onSurface",
"md.comp.full-screen-dialog.header.icon.size": 24.0,
"md.comp.full-screen-dialog.header.on-scroll.container.color": "surfaceContainer",
"md.comp.full-screen-dialog.header.on-scroll.container.elevation": "md.sys.elevation.level2"
}
| flutter/dev/tools/gen_defaults/data/dialog_fullscreen.json/0 | {
"file_path": "flutter/dev/tools/gen_defaults/data/dialog_fullscreen.json",
"repo_id": "flutter",
"token_count": 707
} | 537 |
{
"version": "v0_206",
"md.comp.navigation-rail.active.focus.icon.color": "onSecondaryContainer",
"md.comp.navigation-rail.active.focus.label-text.color": "onSurface",
"md.comp.navigation-rail.active.focus.state-layer.color": "onSurface",
"md.comp.navigation-rail.active.hover.icon.color": "onSecondaryContainer",
"md.comp.navigation-rail.active.hover.label-text.color": "onSurface",
"md.comp.navigation-rail.active.hover.state-layer.color": "onSurface",
"md.comp.navigation-rail.active.icon.color": "onSecondaryContainer",
"md.comp.navigation-rail.active-indicator.color": "secondaryContainer",
"md.comp.navigation-rail.active-indicator.height": 32.0,
"md.comp.navigation-rail.active-indicator.shape": "md.sys.shape.corner.full",
"md.comp.navigation-rail.active-indicator.width": 56.0,
"md.comp.navigation-rail.active.label-text.color": "onSurface",
"md.comp.navigation-rail.active.pressed.icon.color": "onSecondaryContainer",
"md.comp.navigation-rail.active.pressed.label-text.color": "onSurface",
"md.comp.navigation-rail.active.pressed.state-layer.color": "onSurface",
"md.comp.navigation-rail.container.color": "surface",
"md.comp.navigation-rail.container.elevation": "md.sys.elevation.level0",
"md.comp.navigation-rail.container.shape": "md.sys.shape.corner.none",
"md.comp.navigation-rail.container.width": 80.0,
"md.comp.navigation-rail.focus.state-layer.opacity": "md.sys.state.focus.state-layer-opacity",
"md.comp.navigation-rail.hover.state-layer.opacity": "md.sys.state.hover.state-layer-opacity",
"md.comp.navigation-rail.icon.size": 24.0,
"md.comp.navigation-rail.inactive.focus.icon.color": "onSurface",
"md.comp.navigation-rail.inactive.focus.label-text.color": "onSurface",
"md.comp.navigation-rail.inactive.focus.state-layer.color": "onSurface",
"md.comp.navigation-rail.inactive.hover.icon.color": "onSurface",
"md.comp.navigation-rail.inactive.hover.label-text.color": "onSurface",
"md.comp.navigation-rail.inactive.hover.state-layer.color": "onSurface",
"md.comp.navigation-rail.inactive.icon.color": "onSurfaceVariant",
"md.comp.navigation-rail.inactive.label-text.color": "onSurfaceVariant",
"md.comp.navigation-rail.inactive.pressed.icon.color": "onSurface",
"md.comp.navigation-rail.inactive.pressed.label-text.color": "onSurface",
"md.comp.navigation-rail.inactive.pressed.state-layer.color": "onSurface",
"md.comp.navigation-rail.label-text.text-style": "labelMedium",
"md.comp.navigation-rail.no-label.active-indicator.height": 56.0,
"md.comp.navigation-rail.no-label.active-indicator.shape": "md.sys.shape.corner.full",
"md.comp.navigation-rail.pressed.state-layer.opacity": "md.sys.state.pressed.state-layer-opacity"
}
| flutter/dev/tools/gen_defaults/data/navigation_rail.json/0 | {
"file_path": "flutter/dev/tools/gen_defaults/data/navigation_rail.json",
"repo_id": "flutter",
"token_count": 1018
} | 538 |
{
"version": "v0_206",
"md.comp.filled-text-field.active-indicator.color": "onSurfaceVariant",
"md.comp.filled-text-field.active-indicator.height": 1.0,
"md.comp.filled-text-field.caret.color": "primary",
"md.comp.filled-text-field.container.color": "surfaceContainerHighest",
"md.comp.filled-text-field.container.shape": "md.sys.shape.corner.extra-small.top",
"md.comp.filled-text-field.disabled.active-indicator.color": "onSurface",
"md.comp.filled-text-field.disabled.active-indicator.height": 1.0,
"md.comp.filled-text-field.disabled.active-indicator.opacity": 0.38,
"md.comp.filled-text-field.disabled.container.color": "onSurface",
"md.comp.filled-text-field.disabled.container.opacity": 0.04,
"md.comp.filled-text-field.disabled.input-text.color": "onSurface",
"md.comp.filled-text-field.disabled.input-text.opacity": 0.38,
"md.comp.filled-text-field.disabled.label-text.color": "onSurface",
"md.comp.filled-text-field.disabled.label-text.opacity": 0.38,
"md.comp.filled-text-field.disabled.leading-icon.color": "onSurface",
"md.comp.filled-text-field.disabled.leading-icon.opacity": 0.38,
"md.comp.filled-text-field.disabled.supporting-text.color": "onSurface",
"md.comp.filled-text-field.disabled.supporting-text.opacity": 0.38,
"md.comp.filled-text-field.disabled.trailing-icon.color": "onSurface",
"md.comp.filled-text-field.disabled.trailing-icon.opacity": 0.38,
"md.comp.filled-text-field.error.active-indicator.color": "error",
"md.comp.filled-text-field.error.focus.active-indicator.color": "error",
"md.comp.filled-text-field.error.focus.caret.color": "error",
"md.comp.filled-text-field.error.focus.input-text.color": "onSurface",
"md.comp.filled-text-field.error.focus.label-text.color": "error",
"md.comp.filled-text-field.error.focus.leading-icon.color": "onSurfaceVariant",
"md.comp.filled-text-field.error.focus.supporting-text.color": "error",
"md.comp.filled-text-field.error.focus.trailing-icon.color": "error",
"md.comp.filled-text-field.error.hover.active-indicator.color": "onErrorContainer",
"md.comp.filled-text-field.error.hover.input-text.color": "onSurface",
"md.comp.filled-text-field.error.hover.label-text.color": "onErrorContainer",
"md.comp.filled-text-field.error.hover.leading-icon.color": "onSurfaceVariant",
"md.comp.filled-text-field.error.hover.state-layer.color": "onSurface",
"md.comp.filled-text-field.error.hover.state-layer.opacity": "md.sys.state.hover.state-layer-opacity",
"md.comp.filled-text-field.error.hover.supporting-text.color": "error",
"md.comp.filled-text-field.error.hover.trailing-icon.color": "onErrorContainer",
"md.comp.filled-text-field.error.input-text.color": "onSurface",
"md.comp.filled-text-field.error.label-text.color": "error",
"md.comp.filled-text-field.error.leading-icon.color": "onSurfaceVariant",
"md.comp.filled-text-field.error.supporting-text.color": "error",
"md.comp.filled-text-field.error.trailing-icon.color": "error",
"md.comp.filled-text-field.focus.active-indicator.color": "primary",
"md.comp.filled-text-field.focus.active-indicator.height": 2.0,
"md.comp.filled-text-field.focus.active-indicator.thickness": "md.sys.state.focus-indicator.thickness",
"md.comp.filled-text-field.focus.input-text.color": "onSurface",
"md.comp.filled-text-field.focus.label-text.color": "primary",
"md.comp.filled-text-field.focus.leading-icon.color": "onSurfaceVariant",
"md.comp.filled-text-field.focus.supporting-text.color": "onSurfaceVariant",
"md.comp.filled-text-field.focus.trailing-icon.color": "onSurfaceVariant",
"md.comp.filled-text-field.hover.active-indicator.color": "onSurface",
"md.comp.filled-text-field.hover.active-indicator.height": 1.0,
"md.comp.filled-text-field.hover.input-text.color": "onSurface",
"md.comp.filled-text-field.hover.label-text.color": "onSurfaceVariant",
"md.comp.filled-text-field.hover.leading-icon.color": "onSurfaceVariant",
"md.comp.filled-text-field.hover.state-layer.color": "onSurface",
"md.comp.filled-text-field.hover.state-layer.opacity": "md.sys.state.hover.state-layer-opacity",
"md.comp.filled-text-field.hover.supporting-text.color": "onSurfaceVariant",
"md.comp.filled-text-field.hover.trailing-icon.color": "onSurfaceVariant",
"md.comp.filled-text-field.input-text.color": "onSurface",
"md.comp.filled-text-field.input-text.text-style": "bodyLarge",
"md.comp.filled-text-field.input-text.placeholder.color": "onSurfaceVariant",
"md.comp.filled-text-field.input-text.prefix.color": "onSurfaceVariant",
"md.comp.filled-text-field.input-text.suffix.color": "onSurfaceVariant",
"md.comp.filled-text-field.label-text.color": "onSurfaceVariant",
"md.comp.filled-text-field.label-text.text-style": "bodyLarge",
"md.comp.filled-text-field.leading-icon.color": "onSurfaceVariant",
"md.comp.filled-text-field.leading-icon.size": 24.0,
"md.comp.filled-text-field.supporting-text.color": "onSurfaceVariant",
"md.comp.filled-text-field.supporting-text.text-style": "bodySmall",
"md.comp.filled-text-field.trailing-icon.color": "onSurfaceVariant",
"md.comp.filled-text-field.trailing-icon.size": 24.0
}
| flutter/dev/tools/gen_defaults/data/text_field_filled.json/0 | {
"file_path": "flutter/dev/tools/gen_defaults/data/text_field_filled.json",
"repo_id": "flutter",
"token_count": 1939
} | 539 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'template.dart';
class CardTemplate extends TokenTemplate {
const CardTemplate(this.tokenGroup, super.blockName, super.fileName, super.tokens, {
super.colorSchemePrefix = '_colors.',
});
final String tokenGroup;
String _shape() {
final String cardShape = shape('$tokenGroup.container');
if (tokenAvailable('$tokenGroup.outline.color')) {
return '''
$cardShape.copyWith(
side: ${border('$tokenGroup.outline')}
)''';
} else {
return cardShape;
}
}
@override
String generate() => '''
class _${blockName}DefaultsM3 extends CardTheme {
_${blockName}DefaultsM3(this.context)
: super(
clipBehavior: Clip.none,
elevation: ${elevation('$tokenGroup.container')},
margin: const EdgeInsets.all(4.0),
);
final BuildContext context;
late final ColorScheme _colors = Theme.of(context).colorScheme;
@override
Color? get color => ${componentColor('$tokenGroup.container')};
@override
Color? get shadowColor => ${colorOrTransparent('$tokenGroup.container.shadow-color')};
@override
Color? get surfaceTintColor => ${colorOrTransparent('$tokenGroup.container.surface-tint-layer.color')};
@override
ShapeBorder? get shape =>${_shape()};
}
''';
}
| flutter/dev/tools/gen_defaults/lib/card_template.dart/0 | {
"file_path": "flutter/dev/tools/gen_defaults/lib/card_template.dart",
"repo_id": "flutter",
"token_count": 490
} | 540 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'template.dart';
import 'token_logger.dart';
class MotionTemplate extends TokenTemplate {
/// Since we generate the tokens dynamically, we need to store them and log
/// them manually, instead of using [getToken].
MotionTemplate(String blockName, String fileName, this.tokens, this.tokensLogger) : super(blockName, fileName, tokens);
Map<String, dynamic> tokens;
TokenLogger tokensLogger;
// List of duration tokens.
late List<MapEntry<String, dynamic>> durationTokens = tokens.entries.where(
(MapEntry<String, dynamic> entry) => entry.key.contains('.duration.')
).toList()
..sort(
(MapEntry<String, dynamic> a, MapEntry<String, dynamic> b) => (a.value as double).compareTo(b.value as double)
);
// List of easing curve tokens.
late List<MapEntry<String, dynamic>> easingCurveTokens = tokens.entries.where(
(MapEntry<String, dynamic> entry) => entry.key.contains('.easing.')
).toList()
..sort(
// Sort the legacy curves at the end of the list.
(MapEntry<String, dynamic> a, MapEntry<String, dynamic> b) => a.key.contains('legacy') ? 1 : a.key.compareTo(b.key)
);
String durationTokenString(String token, dynamic tokenValue) {
tokensLogger.log(token);
final String tokenName = token.split('.').last.replaceAll('-', '').replaceFirst('Ms', '');
final int milliseconds = (tokenValue as double).toInt();
return
'''
/// The $tokenName duration (${milliseconds}ms) in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Duration tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#c009dec6-f29b-4503-b9f0-482af14a8bbd)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Duration $tokenName = Duration(milliseconds: $milliseconds);
''';
}
String easingCurveTokenString(String token, dynamic tokenValue) {
tokensLogger.log(token);
final String tokenName = token
.replaceFirst('md.sys.motion.easing.', '')
.replaceAllMapped(RegExp(r'[-\.](\w)'), (Match match) {
return match.group(1)!.toUpperCase();
});
return '''
/// The $tokenName easing curve in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Easing tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#433b1153-2ea3-4fe2-9748-803a47bc97ee)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
static const Curve $tokenName = $tokenValue;
''';
}
@override
String generate() => '''
/// The set of durations in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Duration tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#c009dec6-f29b-4503-b9f0-482af14a8bbd)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
abstract final class Durations {
${durationTokens.map((MapEntry<String, dynamic> entry) => durationTokenString(entry.key, entry.value)).join('\n')}}
// TODO(guidezpl): Improve with description and assets, b/289870605
/// The set of easing curves in the Material specification.
///
/// See also:
///
/// * [M3 guidelines: Easing tokens](https://m3.material.io/styles/motion/easing-and-duration/tokens-specs#433b1153-2ea3-4fe2-9748-803a47bc97ee)
/// * [M3 guidelines: Applying easing and duration](https://m3.material.io/styles/motion/easing-and-duration/applying-easing-and-duration)
/// * [Curves], for a collection of non-Material animation easing curves.
abstract final class Easing {
${easingCurveTokens.map((MapEntry<String, dynamic> entry) => easingCurveTokenString(entry.key, entry.value)).join('\n')}}
''';
}
| flutter/dev/tools/gen_defaults/lib/motion_template.dart/0 | {
"file_path": "flutter/dev/tools/gen_defaults/lib/motion_template.dart",
"repo_id": "flutter",
"token_count": 1342
} | 541 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'template.dart';
class TextFieldTemplate extends TokenTemplate {
const TextFieldTemplate(super.blockName, super.fileName, super.tokens);
@override
String generate() => '''
TextStyle? _m3StateInputStyle(BuildContext context) => MaterialStateTextStyle.resolveWith((Set<MaterialState> states) {
if (states.contains(MaterialState.disabled)) {
return TextStyle(color: ${textStyle("md.comp.filled-text-field.label-text")}!.color?.withOpacity(0.38));
}
return TextStyle(color: ${textStyle("md.comp.filled-text-field.label-text")}!.color);
});
TextStyle _m3InputStyle(BuildContext context) => ${textStyle("md.comp.filled-text-field.label-text")}!;
TextStyle _m3CounterErrorStyle(BuildContext context) =>
${textStyle("md.comp.filled-text-field.supporting-text")}!.copyWith(color: ${componentColor('md.comp.filled-text-field.error.supporting-text')});
''';
}
| flutter/dev/tools/gen_defaults/lib/text_field_template.dart/0 | {
"file_path": "flutter/dev/tools/gen_defaults/lib/text_field_template.dart",
"repo_id": "flutter",
"token_count": 327
} | 542 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#include <map>
// DO NOT EDIT -- DO NOT EDIT -- DO NOT EDIT
// This file is generated by
// flutter/flutter:dev/tools/gen_keycodes/bin/gen_keycodes.dart and should not
// be edited directly.
//
// Edit the template
// flutter/flutter:dev/tools/gen_keycodes/data/glfw_keyboard_map_cc.tmpl
// instead.
//
// See flutter/flutter:dev/tools/gen_keycodes/README.md for more information.
/// Maps GLFW-specific key codes to the matching [LogicalKeyboardKey].
const std::map<int, int> g_glfw_to_logical_key = {
@@@GLFW_KEY_CODE_MAP@@@
};
/// A map of GLFW key codes which have printable representations, but appear
/// on the number pad. Used to provide different key objects for keys like
/// KEY_EQUALS and NUMPAD_EQUALS.
const std::map<int, int> g_glfw_numpad_map = {
@@@GLFW_NUMPAD_MAP@@@
};
| flutter/dev/tools/gen_keycodes/data/glfw_keyboard_map_cc.tmpl/0 | {
"file_path": "flutter/dev/tools/gen_keycodes/data/glfw_keyboard_map_cc.tmpl",
"repo_id": "flutter",
"token_count": 323
} | 543 |
{
"Hyper": {
"names": {
"name": "Hyper",
"chromium": "Hyper"
},
"scanCodes": {
"usb": 16
}
},
"Super": {
"names": {
"name": "Super",
"chromium": "Super"
},
"scanCodes": {
"usb": 17
}
},
"Fn": {
"names": {
"name": "Fn",
"chromium": "Fn"
},
"scanCodes": {
"android": [
464
],
"usb": 18,
"macos": 63
}
},
"FnLock": {
"names": {
"name": "FnLock",
"chromium": "FnLock"
},
"scanCodes": {
"usb": 19
}
},
"Suspend": {
"names": {
"name": "Suspend",
"chromium": "Suspend"
},
"scanCodes": {
"android": [
205
],
"usb": 20
}
},
"Resume": {
"names": {
"name": "Resume",
"chromium": "Resume"
},
"scanCodes": {
"usb": 21
}
},
"Turbo": {
"names": {
"name": "Turbo",
"chromium": "Turbo"
},
"scanCodes": {
"usb": 22
}
},
"PrivacyScreenToggle": {
"names": {
"name": "PrivacyScreenToggle",
"chromium": "PrivacyScreenToggle"
},
"scanCodes": {
"usb": 23,
"linux": 633,
"xkb": 641
}
},
"MicrophoneMuteToggle": {
"names": {
"name": "MicrophoneMuteToggle",
"chromium": "MicrophoneMuteToggle"
},
"scanCodes": {
"usb": 24,
"linux": 248,
"xkb": 256
}
},
"Sleep": {
"names": {
"name": "Sleep",
"chromium": "Sleep"
},
"scanCodes": {
"android": [
142
],
"usb": 65666,
"linux": 142,
"xkb": 150,
"windows": 57439
}
},
"WakeUp": {
"names": {
"name": "WakeUp",
"chromium": "WakeUp"
},
"scanCodes": {
"android": [
143
],
"usb": 65667,
"linux": 143,
"xkb": 151,
"windows": 57443
}
},
"DisplayToggleIntExt": {
"names": {
"name": "DisplayToggleIntExt",
"chromium": "DisplayToggleIntExt"
},
"scanCodes": {
"usb": 65717,
"linux": 227,
"xkb": 235
}
},
"GameButton1": {
"names": {
"name": "GameButton1",
"chromium": "GameButton1"
},
"scanCodes": {
"android": [
256,
288
],
"usb": 392961
}
},
"GameButton2": {
"names": {
"name": "GameButton2",
"chromium": "GameButton2"
},
"scanCodes": {
"android": [
257,
289
],
"usb": 392962
}
},
"GameButton3": {
"names": {
"name": "GameButton3",
"chromium": "GameButton3"
},
"scanCodes": {
"android": [
258,
290
],
"usb": 392963
}
},
"GameButton4": {
"names": {
"name": "GameButton4",
"chromium": "GameButton4"
},
"scanCodes": {
"android": [
259,
291
],
"usb": 392964
}
},
"GameButton5": {
"names": {
"name": "GameButton5",
"chromium": "GameButton5"
},
"scanCodes": {
"android": [
260,
292
],
"usb": 392965
}
},
"GameButton6": {
"names": {
"name": "GameButton6",
"chromium": "GameButton6"
},
"scanCodes": {
"android": [
261,
293
],
"usb": 392966
}
},
"GameButton7": {
"names": {
"name": "GameButton7",
"chromium": "GameButton7"
},
"scanCodes": {
"android": [
262,
294
],
"usb": 392967
}
},
"GameButton8": {
"names": {
"name": "GameButton8",
"chromium": "GameButton8"
},
"scanCodes": {
"android": [
263,
295
],
"usb": 392968
}
},
"GameButton9": {
"names": {
"name": "GameButton9",
"chromium": "GameButton9"
},
"scanCodes": {
"android": [
264,
296
],
"usb": 392969
}
},
"GameButton10": {
"names": {
"name": "GameButton10",
"chromium": "GameButton10"
},
"scanCodes": {
"android": [
265,
297
],
"usb": 392970
}
},
"GameButton11": {
"names": {
"name": "GameButton11",
"chromium": "GameButton11"
},
"scanCodes": {
"android": [
266,
298
],
"usb": 392971
}
},
"GameButton12": {
"names": {
"name": "GameButton12",
"chromium": "GameButton12"
},
"scanCodes": {
"android": [
267,
299
],
"usb": 392972
}
},
"GameButton13": {
"names": {
"name": "GameButton13",
"chromium": "GameButton13"
},
"scanCodes": {
"android": [
268,
300
],
"usb": 392973
}
},
"GameButton14": {
"names": {
"name": "GameButton14",
"chromium": "GameButton14"
},
"scanCodes": {
"android": [
269,
301
],
"usb": 392974
}
},
"GameButton15": {
"names": {
"name": "GameButton15",
"chromium": "GameButton15"
},
"scanCodes": {
"android": [
270,
302
],
"usb": 392975
}
},
"GameButton16": {
"names": {
"name": "GameButton16",
"chromium": "GameButton16"
},
"scanCodes": {
"android": [
271,
303
],
"usb": 392976
}
},
"GameButtonA": {
"names": {
"name": "GameButtonA",
"chromium": "GameButtonA"
},
"scanCodes": {
"android": [
304
],
"usb": 392977
}
},
"GameButtonB": {
"names": {
"name": "GameButtonB",
"chromium": "GameButtonB"
},
"scanCodes": {
"android": [
305
],
"usb": 392978
}
},
"GameButtonC": {
"names": {
"name": "GameButtonC",
"chromium": "GameButtonC"
},
"scanCodes": {
"android": [
306
],
"usb": 392979
}
},
"GameButtonLeft1": {
"names": {
"name": "GameButtonLeft1",
"chromium": "GameButtonLeft1"
},
"scanCodes": {
"android": [
310
],
"usb": 392980
}
},
"GameButtonLeft2": {
"names": {
"name": "GameButtonLeft2",
"chromium": "GameButtonLeft2"
},
"scanCodes": {
"android": [
312
],
"usb": 392981
}
},
"GameButtonMode": {
"names": {
"name": "GameButtonMode",
"chromium": "GameButtonMode"
},
"scanCodes": {
"android": [
316
],
"usb": 392982
}
},
"GameButtonRight1": {
"names": {
"name": "GameButtonRight1",
"chromium": "GameButtonRight1"
},
"scanCodes": {
"android": [
311
],
"usb": 392983
}
},
"GameButtonRight2": {
"names": {
"name": "GameButtonRight2",
"chromium": "GameButtonRight2"
},
"scanCodes": {
"android": [
313
],
"usb": 392984
}
},
"GameButtonSelect": {
"names": {
"name": "GameButtonSelect",
"chromium": "GameButtonSelect"
},
"scanCodes": {
"android": [
314
],
"usb": 392985
}
},
"GameButtonStart": {
"names": {
"name": "GameButtonStart",
"chromium": "GameButtonStart"
},
"scanCodes": {
"android": [
315
],
"usb": 392986
}
},
"GameButtonThumbLeft": {
"names": {
"name": "GameButtonThumbLeft",
"chromium": "GameButtonThumbLeft"
},
"scanCodes": {
"android": [
317
],
"usb": 392987
}
},
"GameButtonThumbRight": {
"names": {
"name": "GameButtonThumbRight",
"chromium": "GameButtonThumbRight"
},
"scanCodes": {
"android": [
318
],
"usb": 392988
}
},
"GameButtonX": {
"names": {
"name": "GameButtonX",
"chromium": "GameButtonX"
},
"scanCodes": {
"android": [
307
],
"usb": 392989
}
},
"GameButtonY": {
"names": {
"name": "GameButtonY",
"chromium": "GameButtonY"
},
"scanCodes": {
"android": [
308
],
"usb": 392990
}
},
"GameButtonZ": {
"names": {
"name": "GameButtonZ",
"chromium": "GameButtonZ"
},
"scanCodes": {
"android": [
309
],
"usb": 392991
}
},
"UsbReserved": {
"names": {
"name": "UsbReserved"
},
"scanCodes": {
"usb": 458752,
"ios": 0
}
},
"UsbErrorRollOver": {
"names": {
"name": "UsbErrorRollOver"
},
"scanCodes": {
"usb": 458753,
"windows": 255,
"ios": 1
}
},
"UsbPostFail": {
"names": {
"name": "UsbPostFail"
},
"scanCodes": {
"usb": 458754,
"windows": 252,
"ios": 2
}
},
"UsbErrorUndefined": {
"names": {
"name": "UsbErrorUndefined"
},
"scanCodes": {
"usb": 458755,
"ios": 3
}
},
"KeyA": {
"names": {
"name": "KeyA",
"chromium": "KeyA"
},
"scanCodes": {
"android": [
30
],
"usb": 458756,
"linux": 30,
"xkb": 38,
"windows": 30,
"macos": 0,
"ios": 4
}
},
"KeyB": {
"names": {
"name": "KeyB",
"chromium": "KeyB"
},
"scanCodes": {
"android": [
48
],
"usb": 458757,
"linux": 48,
"xkb": 56,
"windows": 48,
"macos": 11,
"ios": 5
}
},
"KeyC": {
"names": {
"name": "KeyC",
"chromium": "KeyC"
},
"scanCodes": {
"android": [
46
],
"usb": 458758,
"linux": 46,
"xkb": 54,
"windows": 46,
"macos": 8,
"ios": 6
}
},
"KeyD": {
"names": {
"name": "KeyD",
"chromium": "KeyD"
},
"scanCodes": {
"android": [
32
],
"usb": 458759,
"linux": 32,
"xkb": 40,
"windows": 32,
"macos": 2,
"ios": 7
}
},
"KeyE": {
"names": {
"name": "KeyE",
"chromium": "KeyE"
},
"scanCodes": {
"android": [
18
],
"usb": 458760,
"linux": 18,
"xkb": 26,
"windows": 18,
"macos": 14,
"ios": 8
}
},
"KeyF": {
"names": {
"name": "KeyF",
"chromium": "KeyF"
},
"scanCodes": {
"android": [
33
],
"usb": 458761,
"linux": 33,
"xkb": 41,
"windows": 33,
"macos": 3,
"ios": 9
}
},
"KeyG": {
"names": {
"name": "KeyG",
"chromium": "KeyG"
},
"scanCodes": {
"android": [
34
],
"usb": 458762,
"linux": 34,
"xkb": 42,
"windows": 34,
"macos": 5,
"ios": 10
}
},
"KeyH": {
"names": {
"name": "KeyH",
"chromium": "KeyH"
},
"scanCodes": {
"android": [
35
],
"usb": 458763,
"linux": 35,
"xkb": 43,
"windows": 35,
"macos": 4,
"ios": 11
}
},
"KeyI": {
"names": {
"name": "KeyI",
"chromium": "KeyI"
},
"scanCodes": {
"android": [
23
],
"usb": 458764,
"linux": 23,
"xkb": 31,
"windows": 23,
"macos": 34,
"ios": 12
}
},
"KeyJ": {
"names": {
"name": "KeyJ",
"chromium": "KeyJ"
},
"scanCodes": {
"android": [
36
],
"usb": 458765,
"linux": 36,
"xkb": 44,
"windows": 36,
"macos": 38,
"ios": 13
}
},
"KeyK": {
"names": {
"name": "KeyK",
"chromium": "KeyK"
},
"scanCodes": {
"android": [
37
],
"usb": 458766,
"linux": 37,
"xkb": 45,
"windows": 37,
"macos": 40,
"ios": 14
}
},
"KeyL": {
"names": {
"name": "KeyL",
"chromium": "KeyL"
},
"scanCodes": {
"android": [
38
],
"usb": 458767,
"linux": 38,
"xkb": 46,
"windows": 38,
"macos": 37,
"ios": 15
}
},
"KeyM": {
"names": {
"name": "KeyM",
"chromium": "KeyM"
},
"scanCodes": {
"android": [
50
],
"usb": 458768,
"linux": 50,
"xkb": 58,
"windows": 50,
"macos": 46,
"ios": 16
}
},
"KeyN": {
"names": {
"name": "KeyN",
"chromium": "KeyN"
},
"scanCodes": {
"android": [
49
],
"usb": 458769,
"linux": 49,
"xkb": 57,
"windows": 49,
"macos": 45,
"ios": 17
}
},
"KeyO": {
"names": {
"name": "KeyO",
"chromium": "KeyO"
},
"scanCodes": {
"android": [
24
],
"usb": 458770,
"linux": 24,
"xkb": 32,
"windows": 24,
"macos": 31,
"ios": 18
}
},
"KeyP": {
"names": {
"name": "KeyP",
"chromium": "KeyP"
},
"scanCodes": {
"android": [
25
],
"usb": 458771,
"linux": 25,
"xkb": 33,
"windows": 25,
"macos": 35,
"ios": 19
}
},
"KeyQ": {
"names": {
"name": "KeyQ",
"chromium": "KeyQ"
},
"scanCodes": {
"android": [
16
],
"usb": 458772,
"linux": 16,
"xkb": 24,
"windows": 16,
"macos": 12,
"ios": 20
}
},
"KeyR": {
"names": {
"name": "KeyR",
"chromium": "KeyR"
},
"scanCodes": {
"android": [
19
],
"usb": 458773,
"linux": 19,
"xkb": 27,
"windows": 19,
"macos": 15,
"ios": 21
}
},
"KeyS": {
"names": {
"name": "KeyS",
"chromium": "KeyS"
},
"scanCodes": {
"android": [
31
],
"usb": 458774,
"linux": 31,
"xkb": 39,
"windows": 31,
"macos": 1,
"ios": 22
}
},
"KeyT": {
"names": {
"name": "KeyT",
"chromium": "KeyT"
},
"scanCodes": {
"android": [
20
],
"usb": 458775,
"linux": 20,
"xkb": 28,
"windows": 20,
"macos": 17,
"ios": 23
}
},
"KeyU": {
"names": {
"name": "KeyU",
"chromium": "KeyU"
},
"scanCodes": {
"android": [
22
],
"usb": 458776,
"linux": 22,
"xkb": 30,
"windows": 22,
"macos": 32,
"ios": 24
}
},
"KeyV": {
"names": {
"name": "KeyV",
"chromium": "KeyV"
},
"scanCodes": {
"android": [
47
],
"usb": 458777,
"linux": 47,
"xkb": 55,
"windows": 47,
"macos": 9,
"ios": 25
}
},
"KeyW": {
"names": {
"name": "KeyW",
"chromium": "KeyW"
},
"scanCodes": {
"android": [
17
],
"usb": 458778,
"linux": 17,
"xkb": 25,
"windows": 17,
"macos": 13,
"ios": 26
}
},
"KeyX": {
"names": {
"name": "KeyX",
"chromium": "KeyX"
},
"scanCodes": {
"android": [
45
],
"usb": 458779,
"linux": 45,
"xkb": 53,
"windows": 45,
"macos": 7,
"ios": 27
}
},
"KeyY": {
"names": {
"name": "KeyY",
"chromium": "KeyY"
},
"scanCodes": {
"android": [
21
],
"usb": 458780,
"linux": 21,
"xkb": 29,
"windows": 21,
"macos": 16,
"ios": 28
}
},
"KeyZ": {
"names": {
"name": "KeyZ",
"chromium": "KeyZ"
},
"scanCodes": {
"android": [
44
],
"usb": 458781,
"linux": 44,
"xkb": 52,
"windows": 44,
"macos": 6,
"ios": 29
}
},
"Digit1": {
"names": {
"name": "Digit1",
"chromium": "Digit1"
},
"scanCodes": {
"android": [
2
],
"usb": 458782,
"linux": 2,
"xkb": 10,
"windows": 2,
"macos": 18,
"ios": 30
}
},
"Digit2": {
"names": {
"name": "Digit2",
"chromium": "Digit2"
},
"scanCodes": {
"android": [
3
],
"usb": 458783,
"linux": 3,
"xkb": 11,
"windows": 3,
"macos": 19,
"ios": 31
}
},
"Digit3": {
"names": {
"name": "Digit3",
"chromium": "Digit3"
},
"scanCodes": {
"android": [
4
],
"usb": 458784,
"linux": 4,
"xkb": 12,
"windows": 4,
"macos": 20,
"ios": 32
}
},
"Digit4": {
"names": {
"name": "Digit4",
"chromium": "Digit4"
},
"scanCodes": {
"android": [
5
],
"usb": 458785,
"linux": 5,
"xkb": 13,
"windows": 5,
"macos": 21,
"ios": 33
}
},
"Digit5": {
"names": {
"name": "Digit5",
"chromium": "Digit5"
},
"scanCodes": {
"android": [
6
],
"usb": 458786,
"linux": 6,
"xkb": 14,
"windows": 6,
"macos": 23,
"ios": 34
}
},
"Digit6": {
"names": {
"name": "Digit6",
"chromium": "Digit6"
},
"scanCodes": {
"android": [
7
],
"usb": 458787,
"linux": 7,
"xkb": 15,
"windows": 7,
"macos": 22,
"ios": 35
}
},
"Digit7": {
"names": {
"name": "Digit7",
"chromium": "Digit7"
},
"scanCodes": {
"android": [
8
],
"usb": 458788,
"linux": 8,
"xkb": 16,
"windows": 8,
"macos": 26,
"ios": 36
}
},
"Digit8": {
"names": {
"name": "Digit8",
"chromium": "Digit8"
},
"scanCodes": {
"android": [
9
],
"usb": 458789,
"linux": 9,
"xkb": 17,
"windows": 9,
"macos": 28,
"ios": 37
}
},
"Digit9": {
"names": {
"name": "Digit9",
"chromium": "Digit9"
},
"scanCodes": {
"android": [
10
],
"usb": 458790,
"linux": 10,
"xkb": 18,
"windows": 10,
"macos": 25,
"ios": 38
}
},
"Digit0": {
"names": {
"name": "Digit0",
"chromium": "Digit0"
},
"scanCodes": {
"android": [
11
],
"usb": 458791,
"linux": 11,
"xkb": 19,
"windows": 11,
"macos": 29,
"ios": 39
}
},
"Enter": {
"names": {
"name": "Enter",
"chromium": "Enter"
},
"scanCodes": {
"android": [
28
],
"usb": 458792,
"linux": 28,
"xkb": 36,
"windows": 28,
"macos": 36,
"ios": 40
}
},
"Escape": {
"names": {
"name": "Escape",
"chromium": "Escape"
},
"otherWebCodes": [
"Esc"
],
"scanCodes": {
"android": [
1
],
"usb": 458793,
"linux": 1,
"xkb": 9,
"windows": 1,
"macos": 53,
"ios": 41
}
},
"Backspace": {
"names": {
"name": "Backspace",
"chromium": "Backspace"
},
"scanCodes": {
"android": [
14
],
"usb": 458794,
"linux": 14,
"xkb": 22,
"windows": 14,
"macos": 51,
"ios": 42
}
},
"Tab": {
"names": {
"name": "Tab",
"chromium": "Tab"
},
"scanCodes": {
"android": [
15
],
"usb": 458795,
"linux": 15,
"xkb": 23,
"windows": 15,
"macos": 48,
"ios": 43
}
},
"Space": {
"names": {
"name": "Space",
"chromium": "Space"
},
"scanCodes": {
"android": [
57
],
"usb": 458796,
"linux": 57,
"xkb": 65,
"windows": 57,
"macos": 49,
"ios": 44
}
},
"Minus": {
"names": {
"name": "Minus",
"chromium": "Minus"
},
"scanCodes": {
"android": [
12
],
"usb": 458797,
"linux": 12,
"xkb": 20,
"windows": 12,
"macos": 27,
"ios": 45
}
},
"Equal": {
"names": {
"name": "Equal",
"chromium": "Equal"
},
"scanCodes": {
"android": [
13
],
"usb": 458798,
"linux": 13,
"xkb": 21,
"windows": 13,
"macos": 24,
"ios": 46
}
},
"BracketLeft": {
"names": {
"name": "BracketLeft",
"chromium": "BracketLeft"
},
"scanCodes": {
"android": [
26
],
"usb": 458799,
"linux": 26,
"xkb": 34,
"windows": 26,
"macos": 33,
"ios": 47
}
},
"BracketRight": {
"names": {
"name": "BracketRight",
"chromium": "BracketRight"
},
"scanCodes": {
"android": [
27
],
"usb": 458800,
"linux": 27,
"xkb": 35,
"windows": 27,
"macos": 30,
"ios": 48
}
},
"Backslash": {
"names": {
"name": "Backslash",
"chromium": "Backslash"
},
"scanCodes": {
"android": [
43,
86
],
"usb": 458801,
"linux": 43,
"xkb": 51,
"windows": 43,
"macos": 42,
"ios": 49
}
},
"Semicolon": {
"names": {
"name": "Semicolon",
"chromium": "Semicolon"
},
"scanCodes": {
"android": [
39
],
"usb": 458803,
"linux": 39,
"xkb": 47,
"windows": 39,
"macos": 41,
"ios": 51
}
},
"Quote": {
"names": {
"name": "Quote",
"chromium": "Quote"
},
"scanCodes": {
"android": [
40
],
"usb": 458804,
"linux": 40,
"xkb": 48,
"windows": 40,
"macos": 39,
"ios": 52
}
},
"Backquote": {
"names": {
"name": "Backquote",
"chromium": "Backquote"
},
"scanCodes": {
"android": [
41
],
"usb": 458805,
"linux": 41,
"xkb": 49,
"windows": 41,
"macos": 50,
"ios": 53
}
},
"Comma": {
"names": {
"name": "Comma",
"chromium": "Comma"
},
"scanCodes": {
"android": [
51
],
"usb": 458806,
"linux": 51,
"xkb": 59,
"windows": 51,
"macos": 43,
"ios": 54
}
},
"Period": {
"names": {
"name": "Period",
"chromium": "Period"
},
"scanCodes": {
"android": [
52
],
"usb": 458807,
"linux": 52,
"xkb": 60,
"windows": 52,
"macos": 47,
"ios": 55
}
},
"Slash": {
"names": {
"name": "Slash",
"chromium": "Slash"
},
"scanCodes": {
"android": [
53
],
"usb": 458808,
"linux": 53,
"xkb": 61,
"windows": 53,
"macos": 44,
"ios": 56
}
},
"CapsLock": {
"names": {
"name": "CapsLock",
"chromium": "CapsLock"
},
"scanCodes": {
"android": [
58
],
"usb": 458809,
"linux": 58,
"xkb": 66,
"windows": 58,
"macos": 57,
"ios": 57
}
},
"F1": {
"names": {
"name": "F1",
"chromium": "F1"
},
"scanCodes": {
"android": [
59
],
"usb": 458810,
"linux": 59,
"xkb": 67,
"windows": 59,
"macos": 122,
"ios": 58
}
},
"F2": {
"names": {
"name": "F2",
"chromium": "F2"
},
"scanCodes": {
"android": [
60
],
"usb": 458811,
"linux": 60,
"xkb": 68,
"windows": 60,
"macos": 120,
"ios": 59
}
},
"F3": {
"names": {
"name": "F3",
"chromium": "F3"
},
"scanCodes": {
"android": [
61
],
"usb": 458812,
"linux": 61,
"xkb": 69,
"windows": 61,
"macos": 99,
"ios": 60
}
},
"F4": {
"names": {
"name": "F4",
"chromium": "F4"
},
"scanCodes": {
"android": [
62
],
"usb": 458813,
"linux": 62,
"xkb": 70,
"windows": 62,
"macos": 118,
"ios": 61
}
},
"F5": {
"names": {
"name": "F5",
"chromium": "F5"
},
"scanCodes": {
"android": [
63
],
"usb": 458814,
"linux": 63,
"xkb": 71,
"windows": 63,
"macos": 96,
"ios": 62
}
},
"F6": {
"names": {
"name": "F6",
"chromium": "F6"
},
"scanCodes": {
"android": [
64
],
"usb": 458815,
"linux": 64,
"xkb": 72,
"windows": 64,
"macos": 97,
"ios": 63
}
},
"F7": {
"names": {
"name": "F7",
"chromium": "F7"
},
"scanCodes": {
"android": [
65
],
"usb": 458816,
"linux": 65,
"xkb": 73,
"windows": 65,
"macos": 98,
"ios": 64
}
},
"F8": {
"names": {
"name": "F8",
"chromium": "F8"
},
"scanCodes": {
"android": [
66
],
"usb": 458817,
"linux": 66,
"xkb": 74,
"windows": 66,
"macos": 100,
"ios": 65
}
},
"F9": {
"names": {
"name": "F9",
"chromium": "F9"
},
"scanCodes": {
"android": [
67
],
"usb": 458818,
"linux": 67,
"xkb": 75,
"windows": 67,
"macos": 101,
"ios": 66
}
},
"F10": {
"names": {
"name": "F10",
"chromium": "F10"
},
"scanCodes": {
"android": [
68
],
"usb": 458819,
"linux": 68,
"xkb": 76,
"windows": 68,
"macos": 109,
"ios": 67
}
},
"F11": {
"names": {
"name": "F11",
"chromium": "F11"
},
"scanCodes": {
"android": [
87
],
"usb": 458820,
"linux": 87,
"xkb": 95,
"windows": 87,
"macos": 103,
"ios": 68
}
},
"F12": {
"names": {
"name": "F12",
"chromium": "F12"
},
"scanCodes": {
"android": [
88
],
"usb": 458821,
"linux": 88,
"xkb": 96,
"windows": 88,
"macos": 111,
"ios": 69
}
},
"PrintScreen": {
"names": {
"name": "PrintScreen",
"chromium": "PrintScreen"
},
"scanCodes": {
"android": [
99
],
"usb": 458822,
"linux": 99,
"xkb": 107,
"windows": 57399,
"ios": 70
}
},
"ScrollLock": {
"names": {
"name": "ScrollLock",
"chromium": "ScrollLock"
},
"scanCodes": {
"android": [
70
],
"usb": 458823,
"linux": 70,
"xkb": 78,
"windows": 70,
"ios": 71
}
},
"Pause": {
"names": {
"name": "Pause",
"chromium": "Pause"
},
"scanCodes": {
"android": [
119,
411
],
"usb": 458824,
"linux": 119,
"xkb": 127,
"windows": 69,
"ios": 72
}
},
"Insert": {
"names": {
"name": "Insert",
"chromium": "Insert"
},
"scanCodes": {
"android": [
110
],
"usb": 458825,
"linux": 110,
"xkb": 118,
"windows": 57426,
"macos": 114,
"ios": 73
}
},
"Home": {
"names": {
"name": "Home",
"chromium": "Home"
},
"scanCodes": {
"android": [
102
],
"usb": 458826,
"linux": 102,
"xkb": 110,
"windows": 57415,
"macos": 115,
"ios": 74
}
},
"PageUp": {
"names": {
"name": "PageUp",
"chromium": "PageUp"
},
"scanCodes": {
"android": [
104,
177
],
"usb": 458827,
"linux": 104,
"xkb": 112,
"windows": 57417,
"macos": 116,
"ios": 75
}
},
"Delete": {
"names": {
"name": "Delete",
"chromium": "Delete"
},
"scanCodes": {
"android": [
111
],
"usb": 458828,
"linux": 111,
"xkb": 119,
"windows": 57427,
"macos": 117,
"ios": 76
}
},
"End": {
"names": {
"name": "End",
"chromium": "End"
},
"scanCodes": {
"android": [
107
],
"usb": 458829,
"linux": 107,
"xkb": 115,
"windows": 57423,
"macos": 119,
"ios": 77
}
},
"PageDown": {
"names": {
"name": "PageDown",
"chromium": "PageDown"
},
"scanCodes": {
"android": [
109,
178
],
"usb": 458830,
"linux": 109,
"xkb": 117,
"windows": 57425,
"macos": 121,
"ios": 78
}
},
"ArrowRight": {
"names": {
"name": "ArrowRight",
"chromium": "ArrowRight"
},
"scanCodes": {
"android": [
106
],
"usb": 458831,
"linux": 106,
"xkb": 114,
"windows": 57421,
"macos": 124,
"ios": 79
}
},
"ArrowLeft": {
"names": {
"name": "ArrowLeft",
"chromium": "ArrowLeft"
},
"scanCodes": {
"android": [
105
],
"usb": 458832,
"linux": 105,
"xkb": 113,
"windows": 57419,
"macos": 123,
"ios": 80
}
},
"ArrowDown": {
"names": {
"name": "ArrowDown",
"chromium": "ArrowDown"
},
"scanCodes": {
"android": [
108
],
"usb": 458833,
"linux": 108,
"xkb": 116,
"windows": 57424,
"macos": 125,
"ios": 81
}
},
"ArrowUp": {
"names": {
"name": "ArrowUp",
"chromium": "ArrowUp"
},
"scanCodes": {
"android": [
103
],
"usb": 458834,
"linux": 103,
"xkb": 111,
"windows": 57416,
"macos": 126,
"ios": 82
}
},
"NumLock": {
"names": {
"name": "NumLock",
"chromium": "NumLock"
},
"scanCodes": {
"android": [
69
],
"usb": 458835,
"linux": 69,
"xkb": 77,
"windows": 57413,
"macos": 71,
"ios": 83
}
},
"NumpadDivide": {
"names": {
"name": "NumpadDivide",
"chromium": "NumpadDivide"
},
"scanCodes": {
"android": [
98
],
"usb": 458836,
"linux": 98,
"xkb": 106,
"windows": 57397,
"macos": 75,
"ios": 84
}
},
"NumpadMultiply": {
"names": {
"name": "NumpadMultiply",
"chromium": "NumpadMultiply"
},
"scanCodes": {
"android": [
55
],
"usb": 458837,
"linux": 55,
"xkb": 63,
"windows": 55,
"macos": 67,
"ios": 85
}
},
"NumpadSubtract": {
"names": {
"name": "NumpadSubtract",
"chromium": "NumpadSubtract"
},
"scanCodes": {
"android": [
74
],
"usb": 458838,
"linux": 74,
"xkb": 82,
"windows": 74,
"macos": 78,
"ios": 86
}
},
"NumpadAdd": {
"names": {
"name": "NumpadAdd",
"chromium": "NumpadAdd"
},
"scanCodes": {
"android": [
78
],
"usb": 458839,
"linux": 78,
"xkb": 86,
"windows": 78,
"macos": 69,
"ios": 87
}
},
"NumpadEnter": {
"names": {
"name": "NumpadEnter",
"chromium": "NumpadEnter"
},
"scanCodes": {
"android": [
96
],
"usb": 458840,
"linux": 96,
"xkb": 104,
"windows": 57372,
"macos": 76,
"ios": 88
}
},
"Numpad1": {
"names": {
"name": "Numpad1",
"chromium": "Numpad1"
},
"scanCodes": {
"android": [
79
],
"usb": 458841,
"linux": 79,
"xkb": 87,
"windows": 79,
"macos": 83,
"ios": 89
}
},
"Numpad2": {
"names": {
"name": "Numpad2",
"chromium": "Numpad2"
},
"scanCodes": {
"android": [
80
],
"usb": 458842,
"linux": 80,
"xkb": 88,
"windows": 80,
"macos": 84,
"ios": 90
}
},
"Numpad3": {
"names": {
"name": "Numpad3",
"chromium": "Numpad3"
},
"scanCodes": {
"android": [
81
],
"usb": 458843,
"linux": 81,
"xkb": 89,
"windows": 81,
"macos": 85,
"ios": 91
}
},
"Numpad4": {
"names": {
"name": "Numpad4",
"chromium": "Numpad4"
},
"scanCodes": {
"android": [
75
],
"usb": 458844,
"linux": 75,
"xkb": 83,
"windows": 75,
"macos": 86,
"ios": 92
}
},
"Numpad5": {
"names": {
"name": "Numpad5",
"chromium": "Numpad5"
},
"scanCodes": {
"android": [
76
],
"usb": 458845,
"linux": 76,
"xkb": 84,
"windows": 76,
"macos": 87,
"ios": 93
}
},
"Numpad6": {
"names": {
"name": "Numpad6",
"chromium": "Numpad6"
},
"scanCodes": {
"android": [
77
],
"usb": 458846,
"linux": 77,
"xkb": 85,
"windows": 77,
"macos": 88,
"ios": 94
}
},
"Numpad7": {
"names": {
"name": "Numpad7",
"chromium": "Numpad7"
},
"scanCodes": {
"android": [
71
],
"usb": 458847,
"linux": 71,
"xkb": 79,
"windows": 71,
"macos": 89,
"ios": 95
}
},
"Numpad8": {
"names": {
"name": "Numpad8",
"chromium": "Numpad8"
},
"scanCodes": {
"android": [
72
],
"usb": 458848,
"linux": 72,
"xkb": 80,
"windows": 72,
"macos": 91,
"ios": 96
}
},
"Numpad9": {
"names": {
"name": "Numpad9",
"chromium": "Numpad9"
},
"scanCodes": {
"android": [
73
],
"usb": 458849,
"linux": 73,
"xkb": 81,
"windows": 73,
"macos": 92,
"ios": 97
}
},
"Numpad0": {
"names": {
"name": "Numpad0",
"chromium": "Numpad0"
},
"scanCodes": {
"android": [
82
],
"usb": 458850,
"linux": 82,
"xkb": 90,
"windows": 82,
"macos": 82,
"ios": 98
}
},
"NumpadDecimal": {
"names": {
"name": "NumpadDecimal",
"chromium": "NumpadDecimal"
},
"scanCodes": {
"android": [
83
],
"usb": 458851,
"linux": 83,
"xkb": 91,
"windows": 83,
"macos": 65,
"ios": 99
}
},
"IntlBackslash": {
"names": {
"name": "IntlBackslash",
"chromium": "IntlBackslash"
},
"scanCodes": {
"usb": 458852,
"linux": 86,
"xkb": 94,
"windows": 86,
"macos": 10,
"ios": 100
}
},
"ContextMenu": {
"names": {
"name": "ContextMenu",
"chromium": "ContextMenu"
},
"scanCodes": {
"android": [
127,
139
],
"usb": 458853,
"linux": 127,
"xkb": 135,
"windows": 57437,
"macos": 110,
"ios": 101
}
},
"Power": {
"names": {
"name": "Power",
"chromium": "Power"
},
"scanCodes": {
"android": [
116,
152
],
"usb": 458854,
"linux": 116,
"xkb": 124,
"windows": 57438,
"ios": 102
}
},
"NumpadEqual": {
"names": {
"name": "NumpadEqual",
"chromium": "NumpadEqual"
},
"scanCodes": {
"android": [
117
],
"usb": 458855,
"linux": 117,
"xkb": 125,
"windows": 89,
"macos": 81,
"ios": 103
}
},
"F13": {
"names": {
"name": "F13",
"chromium": "F13"
},
"scanCodes": {
"android": [
183
],
"usb": 458856,
"linux": 183,
"xkb": 191,
"windows": 100,
"macos": 105,
"ios": 104
}
},
"F14": {
"names": {
"name": "F14",
"chromium": "F14"
},
"scanCodes": {
"android": [
184
],
"usb": 458857,
"linux": 184,
"xkb": 192,
"windows": 101,
"macos": 107,
"ios": 105
}
},
"F15": {
"names": {
"name": "F15",
"chromium": "F15"
},
"scanCodes": {
"android": [
185
],
"usb": 458858,
"linux": 185,
"xkb": 193,
"windows": 102,
"macos": 113,
"ios": 106
}
},
"F16": {
"names": {
"name": "F16",
"chromium": "F16"
},
"scanCodes": {
"android": [
186
],
"usb": 458859,
"linux": 186,
"xkb": 194,
"windows": 103,
"macos": 106,
"ios": 107
}
},
"F17": {
"names": {
"name": "F17",
"chromium": "F17"
},
"scanCodes": {
"android": [
187
],
"usb": 458860,
"linux": 187,
"xkb": 195,
"windows": 104,
"macos": 64,
"ios": 108
}
},
"F18": {
"names": {
"name": "F18",
"chromium": "F18"
},
"scanCodes": {
"android": [
188
],
"usb": 458861,
"linux": 188,
"xkb": 196,
"windows": 105,
"macos": 79,
"ios": 109
}
},
"F19": {
"names": {
"name": "F19",
"chromium": "F19"
},
"scanCodes": {
"android": [
189
],
"usb": 458862,
"linux": 189,
"xkb": 197,
"windows": 106,
"macos": 80,
"ios": 110
}
},
"F20": {
"names": {
"name": "F20",
"chromium": "F20"
},
"scanCodes": {
"android": [
190
],
"usb": 458863,
"linux": 190,
"xkb": 198,
"windows": 107,
"macos": 90,
"ios": 111
}
},
"F21": {
"names": {
"name": "F21",
"chromium": "F21"
},
"scanCodes": {
"android": [
191
],
"usb": 458864,
"linux": 191,
"xkb": 199,
"windows": 108,
"ios": 112
}
},
"F22": {
"names": {
"name": "F22",
"chromium": "F22"
},
"scanCodes": {
"android": [
192
],
"usb": 458865,
"linux": 192,
"xkb": 200,
"windows": 109,
"ios": 113
}
},
"F23": {
"names": {
"name": "F23",
"chromium": "F23"
},
"scanCodes": {
"android": [
193
],
"usb": 458866,
"linux": 193,
"xkb": 201,
"windows": 110,
"ios": 114
}
},
"F24": {
"names": {
"name": "F24",
"chromium": "F24"
},
"scanCodes": {
"android": [
194
],
"usb": 458867,
"linux": 194,
"xkb": 202,
"windows": 118,
"ios": 115
}
},
"Open": {
"names": {
"name": "Open",
"chromium": "Open"
},
"scanCodes": {
"android": [
134
],
"usb": 458868,
"linux": 134,
"xkb": 142,
"ios": 116
}
},
"Help": {
"names": {
"name": "Help",
"chromium": "Help"
},
"scanCodes": {
"android": [
138
],
"usb": 458869,
"linux": 138,
"xkb": 146,
"windows": 57403,
"ios": 117
}
},
"Select": {
"names": {
"name": "Select",
"chromium": "Select"
},
"scanCodes": {
"android": [
353
],
"usb": 458871,
"linux": 132,
"xkb": 140,
"ios": 119
}
},
"Again": {
"names": {
"name": "Again",
"chromium": "Again"
},
"scanCodes": {
"android": [
129
],
"usb": 458873,
"linux": 129,
"xkb": 137,
"ios": 121
}
},
"Undo": {
"names": {
"name": "Undo",
"chromium": "Undo"
},
"scanCodes": {
"android": [
131
],
"usb": 458874,
"linux": 131,
"xkb": 139,
"windows": 57352,
"ios": 122
}
},
"Cut": {
"names": {
"name": "Cut",
"chromium": "Cut"
},
"scanCodes": {
"android": [
137
],
"usb": 458875,
"linux": 137,
"xkb": 145,
"windows": 57367,
"ios": 123
}
},
"Copy": {
"names": {
"name": "Copy",
"chromium": "Copy"
},
"scanCodes": {
"android": [
133
],
"usb": 458876,
"linux": 133,
"xkb": 141,
"windows": 57368,
"ios": 124
}
},
"Paste": {
"names": {
"name": "Paste",
"chromium": "Paste"
},
"scanCodes": {
"android": [
135
],
"usb": 458877,
"linux": 135,
"xkb": 143,
"windows": 57354,
"ios": 125
}
},
"Find": {
"names": {
"name": "Find",
"chromium": "Find"
},
"scanCodes": {
"android": [
136
],
"usb": 458878,
"linux": 136,
"xkb": 144,
"ios": 126
}
},
"AudioVolumeMute": {
"names": {
"name": "AudioVolumeMute",
"chromium": "AudioVolumeMute"
},
"scanCodes": {
"android": [
113
],
"usb": 458879,
"linux": 113,
"xkb": 121,
"windows": 57376,
"macos": 74,
"ios": 127
}
},
"AudioVolumeUp": {
"names": {
"name": "AudioVolumeUp",
"chromium": "AudioVolumeUp"
},
"scanCodes": {
"android": [
115
],
"usb": 458880,
"linux": 115,
"xkb": 123,
"windows": 57392,
"macos": 72,
"ios": 128
}
},
"AudioVolumeDown": {
"names": {
"name": "AudioVolumeDown",
"chromium": "AudioVolumeDown"
},
"scanCodes": {
"android": [
114
],
"usb": 458881,
"linux": 114,
"xkb": 122,
"windows": 57390,
"macos": 73,
"ios": 129
}
},
"NumpadComma": {
"names": {
"name": "NumpadComma",
"chromium": "NumpadComma"
},
"scanCodes": {
"android": [
95,
121
],
"usb": 458885,
"linux": 121,
"xkb": 129,
"windows": 126,
"macos": 95,
"ios": 133
}
},
"IntlRo": {
"names": {
"name": "IntlRo",
"chromium": "IntlRo"
},
"scanCodes": {
"android": [
89
],
"usb": 458887,
"linux": 89,
"xkb": 97,
"windows": 115,
"macos": 94,
"ios": 135
}
},
"KanaMode": {
"names": {
"name": "KanaMode",
"chromium": "KanaMode"
},
"scanCodes": {
"usb": 458888,
"linux": 93,
"xkb": 101,
"windows": 112,
"ios": 136
}
},
"IntlYen": {
"names": {
"name": "IntlYen",
"chromium": "IntlYen"
},
"scanCodes": {
"android": [
124
],
"usb": 458889,
"linux": 124,
"xkb": 132,
"windows": 125,
"macos": 93,
"ios": 137
}
},
"Convert": {
"names": {
"name": "Convert",
"chromium": "Convert"
},
"scanCodes": {
"android": [
92
],
"usb": 458890,
"linux": 92,
"xkb": 100,
"windows": 121,
"ios": 138
}
},
"NonConvert": {
"names": {
"name": "NonConvert",
"chromium": "NonConvert"
},
"scanCodes": {
"android": [
94
],
"usb": 458891,
"linux": 94,
"xkb": 102,
"windows": 123,
"ios": 139
}
},
"Lang1": {
"names": {
"name": "Lang1",
"chromium": "Lang1"
},
"scanCodes": {
"usb": 458896,
"linux": 122,
"xkb": 130,
"windows": 114,
"macos": 104,
"ios": 144
}
},
"Lang2": {
"names": {
"name": "Lang2",
"chromium": "Lang2"
},
"scanCodes": {
"usb": 458897,
"linux": 123,
"xkb": 131,
"windows": 113,
"macos": 102,
"ios": 145
}
},
"Lang3": {
"names": {
"name": "Lang3",
"chromium": "Lang3"
},
"scanCodes": {
"android": [
90
],
"usb": 458898,
"linux": 90,
"xkb": 98,
"windows": 120,
"ios": 146
}
},
"Lang4": {
"names": {
"name": "Lang4",
"chromium": "Lang4"
},
"scanCodes": {
"android": [
91
],
"usb": 458899,
"linux": 91,
"xkb": 99,
"windows": 119,
"ios": 147
}
},
"Lang5": {
"names": {
"name": "Lang5",
"chromium": "Lang5"
},
"scanCodes": {
"usb": 458900,
"linux": 85,
"xkb": 93,
"ios": 148
}
},
"Abort": {
"names": {
"name": "Abort",
"chromium": "Abort"
},
"scanCodes": {
"usb": 458907,
"ios": 155
}
},
"Props": {
"names": {
"name": "Props",
"chromium": "Props"
},
"scanCodes": {
"android": [
130
],
"usb": 458915,
"ios": 163
}
},
"NumpadParenLeft": {
"names": {
"name": "NumpadParenLeft",
"chromium": "NumpadParenLeft"
},
"scanCodes": {
"android": [
179
],
"usb": 458934,
"linux": 179,
"xkb": 187,
"ios": 182
}
},
"NumpadParenRight": {
"names": {
"name": "NumpadParenRight",
"chromium": "NumpadParenRight"
},
"scanCodes": {
"android": [
180
],
"usb": 458935,
"linux": 180,
"xkb": 188,
"ios": 183
}
},
"NumpadBackspace": {
"names": {
"name": "NumpadBackspace",
"chromium": "NumpadBackspace"
},
"scanCodes": {
"usb": 458939,
"ios": 187
}
},
"NumpadMemoryStore": {
"names": {
"name": "NumpadMemoryStore",
"chromium": "NumpadMemoryStore"
},
"scanCodes": {
"usb": 458960,
"ios": 208
}
},
"NumpadMemoryRecall": {
"names": {
"name": "NumpadMemoryRecall",
"chromium": "NumpadMemoryRecall"
},
"scanCodes": {
"usb": 458961,
"ios": 209
}
},
"NumpadMemoryClear": {
"names": {
"name": "NumpadMemoryClear",
"chromium": "NumpadMemoryClear"
},
"scanCodes": {
"usb": 458962,
"ios": 210
}
},
"NumpadMemoryAdd": {
"names": {
"name": "NumpadMemoryAdd",
"chromium": "NumpadMemoryAdd"
},
"scanCodes": {
"usb": 458963,
"ios": 211
}
},
"NumpadMemorySubtract": {
"names": {
"name": "NumpadMemorySubtract",
"chromium": "NumpadMemorySubtract"
},
"scanCodes": {
"usb": 458964,
"ios": 212
}
},
"NumpadSignChange": {
"names": {
"name": "NumpadSignChange"
},
"scanCodes": {
"usb": 458967,
"linux": 118,
"xkb": 126,
"ios": 215
}
},
"NumpadClear": {
"names": {
"name": "NumpadClear",
"chromium": "NumpadClear"
},
"scanCodes": {
"usb": 458968,
"ios": 216
}
},
"NumpadClearEntry": {
"names": {
"name": "NumpadClearEntry",
"chromium": "NumpadClearEntry"
},
"scanCodes": {
"usb": 458969,
"ios": 217
}
},
"ControlLeft": {
"names": {
"name": "ControlLeft",
"chromium": "ControlLeft"
},
"scanCodes": {
"android": [
29
],
"usb": 458976,
"linux": 29,
"xkb": 37,
"windows": 29,
"macos": 59,
"ios": 224
}
},
"ShiftLeft": {
"names": {
"name": "ShiftLeft",
"chromium": "ShiftLeft"
},
"scanCodes": {
"android": [
42
],
"usb": 458977,
"linux": 42,
"xkb": 50,
"windows": 42,
"macos": 56,
"ios": 225
}
},
"AltLeft": {
"names": {
"name": "AltLeft",
"chromium": "AltLeft"
},
"scanCodes": {
"android": [
56
],
"usb": 458978,
"linux": 56,
"xkb": 64,
"windows": 56,
"macos": 58,
"ios": 226
}
},
"MetaLeft": {
"names": {
"name": "MetaLeft",
"chromium": "MetaLeft"
},
"scanCodes": {
"android": [
125
],
"usb": 458979,
"linux": 125,
"xkb": 133,
"windows": 57435,
"macos": 55,
"ios": 227
}
},
"ControlRight": {
"names": {
"name": "ControlRight",
"chromium": "ControlRight"
},
"scanCodes": {
"android": [
97
],
"usb": 458980,
"linux": 97,
"xkb": 105,
"windows": 57373,
"macos": 62,
"ios": 228
}
},
"ShiftRight": {
"names": {
"name": "ShiftRight",
"chromium": "ShiftRight"
},
"scanCodes": {
"android": [
54
],
"usb": 458981,
"linux": 54,
"xkb": 62,
"windows": 54,
"macos": 60,
"ios": 229
}
},
"AltRight": {
"names": {
"name": "AltRight",
"chromium": "AltRight"
},
"scanCodes": {
"android": [
100
],
"usb": 458982,
"linux": 100,
"xkb": 108,
"windows": 57400,
"macos": 61,
"ios": 230
}
},
"MetaRight": {
"names": {
"name": "MetaRight",
"chromium": "MetaRight"
},
"scanCodes": {
"android": [
126
],
"usb": 458983,
"linux": 126,
"xkb": 134,
"windows": 57436,
"macos": 54,
"ios": 231
}
},
"Info": {
"names": {
"name": "Info"
},
"scanCodes": {
"android": [
358
],
"usb": 786528,
"linux": 358,
"xkb": 366
}
},
"ClosedCaptionToggle": {
"names": {
"name": "ClosedCaptionToggle"
},
"scanCodes": {
"android": [
370
],
"usb": 786529,
"linux": 370,
"xkb": 378
}
},
"BrightnessUp": {
"names": {
"name": "BrightnessUp",
"chromium": "BrightnessUp"
},
"scanCodes": {
"android": [
225
],
"usb": 786543,
"linux": 225,
"xkb": 233
}
},
"BrightnessDown": {
"names": {
"name": "BrightnessDown",
"chromium": "BrightnessDown"
},
"scanCodes": {
"android": [
224
],
"usb": 786544,
"linux": 224,
"xkb": 232
}
},
"BrightnessToggle": {
"names": {
"name": "BrightnessToggle"
},
"scanCodes": {
"usb": 786546,
"linux": 431,
"xkb": 439
}
},
"BrightnessMinimum": {
"names": {
"name": "BrightnessMinimum"
},
"scanCodes": {
"usb": 786547,
"linux": 592,
"xkb": 600
}
},
"BrightnessMaximum": {
"names": {
"name": "BrightnessMaximum"
},
"scanCodes": {
"usb": 786548,
"linux": 593,
"xkb": 601
}
},
"BrightnessAuto": {
"names": {
"name": "BrightnessAuto"
},
"scanCodes": {
"usb": 786549,
"linux": 244,
"xkb": 252
}
},
"KbdIllumUp": {
"names": {
"name": "KbdIllumUp"
},
"scanCodes": {
"usb": 786553,
"linux": 230,
"xkb": 238
}
},
"KbdIllumDown": {
"names": {
"name": "KbdIllumDown"
},
"scanCodes": {
"usb": 786554,
"linux": 229,
"xkb": 237
}
},
"MediaLast": {
"names": {
"name": "MediaLast"
},
"scanCodes": {
"android": [
405
],
"usb": 786563,
"linux": 405,
"xkb": 413
}
},
"LaunchPhone": {
"names": {
"name": "LaunchPhone"
},
"scanCodes": {
"usb": 786572,
"linux": 169,
"xkb": 177
}
},
"ProgramGuide": {
"names": {
"name": "ProgramGuide"
},
"scanCodes": {
"usb": 786573,
"linux": 362,
"xkb": 370
}
},
"Exit": {
"names": {
"name": "Exit"
},
"scanCodes": {
"android": [
174
],
"usb": 786580,
"linux": 174,
"xkb": 182
}
},
"ChannelUp": {
"names": {
"name": "ChannelUp"
},
"scanCodes": {
"android": [
402
],
"usb": 786588,
"linux": 410,
"xkb": 418
}
},
"ChannelDown": {
"names": {
"name": "ChannelDown"
},
"scanCodes": {
"android": [
403
],
"usb": 786589,
"linux": 411,
"xkb": 419
}
},
"MediaPlay": {
"names": {
"name": "MediaPlay",
"chromium": "MediaPlay"
},
"scanCodes": {
"android": [
200,
207
],
"usb": 786608,
"linux": 207,
"xkb": 215
}
},
"MediaPause": {
"names": {
"name": "MediaPause",
"chromium": "MediaPause"
},
"scanCodes": {
"android": [
201
],
"usb": 786609,
"linux": 201,
"xkb": 209
}
},
"MediaRecord": {
"names": {
"name": "MediaRecord",
"chromium": "MediaRecord"
},
"scanCodes": {
"android": [
167
],
"usb": 786610,
"linux": 167,
"xkb": 175
}
},
"MediaFastForward": {
"names": {
"name": "MediaFastForward",
"chromium": "MediaFastForward"
},
"scanCodes": {
"android": [
208
],
"usb": 786611,
"linux": 208,
"xkb": 216
}
},
"MediaRewind": {
"names": {
"name": "MediaRewind",
"chromium": "MediaRewind"
},
"scanCodes": {
"android": [
168
],
"usb": 786612,
"linux": 168,
"xkb": 176
}
},
"MediaTrackNext": {
"names": {
"name": "MediaTrackNext",
"chromium": "MediaTrackNext"
},
"scanCodes": {
"android": [
163
],
"usb": 786613,
"linux": 163,
"xkb": 171,
"windows": 57369
}
},
"MediaTrackPrevious": {
"names": {
"name": "MediaTrackPrevious",
"chromium": "MediaTrackPrevious"
},
"scanCodes": {
"android": [
165
],
"usb": 786614,
"linux": 165,
"xkb": 173,
"windows": 57360
}
},
"MediaStop": {
"names": {
"name": "MediaStop",
"chromium": "MediaStop"
},
"scanCodes": {
"android": [
128,
166
],
"usb": 786615,
"linux": 166,
"xkb": 174,
"windows": 57380
}
},
"Eject": {
"names": {
"name": "Eject",
"chromium": "Eject"
},
"scanCodes": {
"android": [
161,
162
],
"usb": 786616,
"linux": 161,
"xkb": 169,
"windows": 57388
}
},
"MediaPlayPause": {
"names": {
"name": "MediaPlayPause",
"chromium": "MediaPlayPause"
},
"scanCodes": {
"android": [
164
],
"usb": 786637,
"linux": 164,
"xkb": 172,
"windows": 57378
}
},
"SpeechInputToggle": {
"names": {
"name": "SpeechInputToggle"
},
"scanCodes": {
"usb": 786639,
"linux": 582,
"xkb": 590
}
},
"BassBoost": {
"names": {
"name": "BassBoost"
},
"scanCodes": {
"android": [
209
],
"usb": 786661,
"linux": 209,
"xkb": 217
}
},
"MediaSelect": {
"names": {
"name": "MediaSelect",
"chromium": "MediaSelect"
},
"scanCodes": {
"usb": 786819,
"linux": 171,
"xkb": 179,
"windows": 57453
}
},
"LaunchWordProcessor": {
"names": {
"name": "LaunchWordProcessor"
},
"scanCodes": {
"usb": 786820,
"linux": 421,
"xkb": 429
}
},
"LaunchSpreadsheet": {
"names": {
"name": "LaunchSpreadsheet"
},
"scanCodes": {
"usb": 786822,
"linux": 423,
"xkb": 431
}
},
"LaunchMail": {
"names": {
"name": "LaunchMail",
"chromium": "LaunchMail"
},
"scanCodes": {
"android": [
155,
215
],
"usb": 786826,
"linux": 155,
"xkb": 163,
"windows": 57452
}
},
"LaunchContacts": {
"names": {
"name": "LaunchContacts"
},
"scanCodes": {
"android": [
429
],
"usb": 786829,
"linux": 429,
"xkb": 437
}
},
"LaunchCalendar": {
"names": {
"name": "LaunchCalendar"
},
"scanCodes": {
"android": [
397
],
"usb": 786830,
"linux": 397,
"xkb": 405
}
},
"LaunchApp2": {
"names": {
"name": "LaunchApp2",
"chromium": "LaunchApp2"
},
"scanCodes": {
"usb": 786834,
"linux": 140,
"xkb": 148,
"windows": 57377
}
},
"LaunchApp1": {
"names": {
"name": "LaunchApp1",
"chromium": "LaunchApp1"
},
"scanCodes": {
"usb": 786836,
"linux": 144,
"xkb": 152,
"windows": 57451
}
},
"LaunchInternetBrowser": {
"names": {
"name": "LaunchInternetBrowser"
},
"scanCodes": {
"usb": 786838,
"linux": 150,
"xkb": 158
}
},
"LogOff": {
"names": {
"name": "LogOff"
},
"scanCodes": {
"usb": 786844,
"linux": 433,
"xkb": 441
}
},
"LockScreen": {
"names": {
"name": "LockScreen"
},
"scanCodes": {
"usb": 786846,
"linux": 152,
"xkb": 160
}
},
"LaunchControlPanel": {
"names": {
"name": "LaunchControlPanel",
"chromium": "LaunchControlPanel"
},
"scanCodes": {
"usb": 786847,
"linux": 579,
"xkb": 587
}
},
"SelectTask": {
"names": {
"name": "SelectTask",
"chromium": "SelectTask"
},
"scanCodes": {
"usb": 786850,
"linux": 580,
"xkb": 588
}
},
"LaunchDocuments": {
"names": {
"name": "LaunchDocuments"
},
"scanCodes": {
"usb": 786855,
"linux": 235,
"xkb": 243
}
},
"SpellCheck": {
"names": {
"name": "SpellCheck"
},
"scanCodes": {
"usb": 786859,
"linux": 432,
"xkb": 440
}
},
"LaunchKeyboardLayout": {
"names": {
"name": "LaunchKeyboardLayout"
},
"scanCodes": {
"usb": 786862,
"linux": 374,
"xkb": 382
}
},
"LaunchScreenSaver": {
"names": {
"name": "LaunchScreenSaver",
"chromium": "LaunchScreenSaver"
},
"scanCodes": {
"usb": 786865,
"linux": 581,
"xkb": 589
}
},
"LaunchAudioBrowser": {
"names": {
"name": "LaunchAudioBrowser"
},
"scanCodes": {
"usb": 786871,
"linux": 392,
"xkb": 400
}
},
"LaunchAssistant": {
"names": {
"name": "LaunchAssistant",
"chromium": "LaunchAssistant"
},
"scanCodes": {
"android": [
583
],
"usb": 786891,
"linux": 583,
"xkb": 591
}
},
"New": {
"names": {
"name": "New"
},
"scanCodes": {
"usb": 786945,
"linux": 181,
"xkb": 189
}
},
"Close": {
"names": {
"name": "Close"
},
"scanCodes": {
"android": [
160,
206
],
"usb": 786947,
"linux": 206,
"xkb": 214
}
},
"Save": {
"names": {
"name": "Save"
},
"scanCodes": {
"usb": 786951,
"linux": 234,
"xkb": 242
}
},
"Print": {
"names": {
"name": "Print"
},
"scanCodes": {
"android": [
210
],
"usb": 786952,
"linux": 210,
"xkb": 218
}
},
"BrowserSearch": {
"names": {
"name": "BrowserSearch",
"chromium": "BrowserSearch"
},
"scanCodes": {
"android": [
217
],
"usb": 786977,
"linux": 217,
"xkb": 225,
"windows": 57445
}
},
"BrowserHome": {
"names": {
"name": "BrowserHome",
"chromium": "BrowserHome"
},
"scanCodes": {
"usb": 786979,
"linux": 172,
"xkb": 180,
"windows": 57394
}
},
"BrowserBack": {
"names": {
"name": "BrowserBack",
"chromium": "BrowserBack"
},
"scanCodes": {
"usb": 786980,
"linux": 158,
"xkb": 166,
"windows": 57450
}
},
"BrowserForward": {
"names": {
"name": "BrowserForward",
"chromium": "BrowserForward"
},
"scanCodes": {
"android": [
159
],
"usb": 786981,
"linux": 159,
"xkb": 167,
"windows": 57449
}
},
"BrowserStop": {
"names": {
"name": "BrowserStop",
"chromium": "BrowserStop"
},
"scanCodes": {
"usb": 786982,
"linux": 128,
"xkb": 136,
"windows": 57448
}
},
"BrowserRefresh": {
"names": {
"name": "BrowserRefresh",
"chromium": "BrowserRefresh"
},
"scanCodes": {
"usb": 786983,
"linux": 173,
"xkb": 181,
"windows": 57447
}
},
"BrowserFavorites": {
"names": {
"name": "BrowserFavorites",
"chromium": "BrowserFavorites"
},
"scanCodes": {
"android": [
156
],
"usb": 786986,
"linux": 156,
"xkb": 164,
"windows": 57446
}
},
"ZoomIn": {
"names": {
"name": "ZoomIn"
},
"scanCodes": {
"usb": 786989,
"linux": 418,
"xkb": 426
}
},
"ZoomOut": {
"names": {
"name": "ZoomOut"
},
"scanCodes": {
"usb": 786990,
"linux": 419,
"xkb": 427
}
},
"ZoomToggle": {
"names": {
"name": "ZoomToggle",
"chromium": "ZoomToggle"
},
"scanCodes": {
"usb": 786994,
"linux": 372,
"xkb": 380
}
},
"Redo": {
"names": {
"name": "Redo"
},
"scanCodes": {
"android": [
182
],
"usb": 787065,
"linux": 182,
"xkb": 190
}
},
"MailReply": {
"names": {
"name": "MailReply",
"chromium": "MailReply"
},
"scanCodes": {
"usb": 787081,
"linux": 232,
"xkb": 240
}
},
"MailForward": {
"names": {
"name": "MailForward",
"chromium": "MailForward"
},
"scanCodes": {
"usb": 787083,
"linux": 233,
"xkb": 241
}
},
"MailSend": {
"names": {
"name": "MailSend",
"chromium": "MailSend"
},
"scanCodes": {
"usb": 787084,
"linux": 231,
"xkb": 239
}
},
"KeyboardLayoutSelect": {
"names": {
"name": "KeyboardLayoutSelect",
"chromium": "KeyboardLayoutSelect"
},
"scanCodes": {
"usb": 787101,
"linux": 584,
"xkb": 592
}
},
"ShowAllWindows": {
"names": {
"name": "ShowAllWindows",
"chromium": "ShowAllWindows"
},
"scanCodes": {
"usb": 787103,
"linux": 120,
"xkb": 128
}
}
}
| flutter/dev/tools/gen_keycodes/data/physical_key_data.g.json/0 | {
"file_path": "flutter/dev/tools/gen_keycodes/data/physical_key_data.g.json",
"repo_id": "flutter",
"token_count": 36267
} | 544 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:path/path.dart' as path;
import 'base_code_gen.dart';
import 'constants.dart';
import 'logical_key_data.dart';
import 'physical_key_data.dart';
import 'utils.dart';
/// Given an [input] string, wraps the text at 80 characters and prepends each
/// line with the [prefix] string. Use for generated comments.
String _wrapString(String input) {
return wrapString(input, prefix: ' /// ');
}
final List<MaskConstant> _maskConstants = <MaskConstant>[
kValueMask,
kPlaneMask,
kUnicodePlane,
kUnprintablePlane,
kFlutterPlane,
kStartOfPlatformPlanes,
kAndroidPlane,
kFuchsiaPlane,
kIosPlane,
kMacosPlane,
kGtkPlane,
kWindowsPlane,
kWebPlane,
kGlfwPlane,
];
class SynonymKeyInfo {
SynonymKeyInfo(this.keys, this.name);
final List<LogicalKeyEntry> keys;
final String name;
// Use the first item in the synonyms as a template for the ID to use.
// It won't end up being the same value because it'll be in the pseudo-key
// plane.
LogicalKeyEntry get primaryKey => keys[0];
String get constantName => upperCamelToLowerCamel(name);
}
/// Generates the keyboard_key.g.dart based on the information in the key data
/// structure given to it.
class KeyboardKeysCodeGenerator extends BaseCodeGenerator {
KeyboardKeysCodeGenerator(super.keyData, super.logicalData);
/// Gets the generated definitions of PhysicalKeyboardKeys.
String get _physicalDefinitions {
final OutputLines<int> lines = OutputLines<int>('Physical Key Definition');
for (final PhysicalKeyEntry entry in keyData.entries) {
final String firstComment = _wrapString('Represents the location of the '
'"${entry.commentName}" key on a generalized keyboard.');
final String otherComments = _wrapString('See the function '
'[RawKeyEvent.physicalKey] for more information.');
lines.add(entry.usbHidCode, '''
$firstComment ///
$otherComments static const PhysicalKeyboardKey ${entry.constantName} = PhysicalKeyboardKey(${toHex(entry.usbHidCode)});
''');
}
return lines.sortedJoin().trimRight();
}
String get _physicalDebugNames {
final OutputLines<int> lines = OutputLines<int>('Physical debug names');
for (final PhysicalKeyEntry entry in keyData.entries) {
lines.add(entry.usbHidCode, '''
${toHex(entry.usbHidCode)}: '${entry.commentName}',''');
}
return lines.sortedJoin().trimRight();
}
/// Gets the generated definitions of LogicalKeyboardKeys.
String get _logicalDefinitions {
final OutputLines<int> lines = OutputLines<int>('Logical debug names', behavior: DeduplicateBehavior.kSkip);
void printKey(int flutterId, String constantName, String commentName, {String? otherComments}) {
final String firstComment = _wrapString('Represents the logical "$commentName" key on the keyboard.');
otherComments ??= _wrapString('See the function [RawKeyEvent.logicalKey] for more information.');
lines.add(flutterId, '''
$firstComment ///
$otherComments static const LogicalKeyboardKey $constantName = LogicalKeyboardKey(${toHex(flutterId, digits: 11)});
''');
}
for (final LogicalKeyEntry entry in logicalData.entries) {
printKey(
entry.value,
entry.constantName,
entry.commentName,
otherComments: _otherComments(entry.name),
);
}
return lines.sortedJoin().trimRight();
}
String? _otherComments(String name) {
if (synonyms.containsKey(name)) {
final Set<String> unionNames = synonyms[name]!.keys.map(
(LogicalKeyEntry entry) => entry.constantName).toSet();
return _wrapString('This key represents the union of the keys '
'$unionNames when comparing keys. This key will never be generated '
'directly, its main use is in defining key maps.');
}
return null;
}
String get _logicalSynonyms {
final StringBuffer result = StringBuffer();
for (final SynonymKeyInfo synonymInfo in synonyms.values) {
for (final LogicalKeyEntry key in synonymInfo.keys) {
final LogicalKeyEntry synonym = logicalData.entryByName(synonymInfo.name);
result.writeln(' ${key.constantName}: <LogicalKeyboardKey>{${synonym.constantName}},');
}
}
return result.toString();
}
String get _logicalReverseSynonyms {
final StringBuffer result = StringBuffer();
for (final SynonymKeyInfo synonymInfo in synonyms.values) {
final LogicalKeyEntry synonym = logicalData.entryByName(synonymInfo.name);
final List<String> entries = synonymInfo.keys.map<String>((LogicalKeyEntry entry) => entry.constantName).toList();
result.writeln(' ${synonym.constantName}: <LogicalKeyboardKey>{${entries.join(', ')}},');
}
return result.toString();
}
String get _logicalKeyLabels {
final OutputLines<int> lines = OutputLines<int>('Logical key labels', behavior: DeduplicateBehavior.kSkip);
for (final LogicalKeyEntry entry in logicalData.entries) {
lines.add(entry.value, '''
${toHex(entry.value, digits: 11)}: '${entry.commentName}',''');
}
return lines.sortedJoin().trimRight();
}
/// This generates the map of USB HID codes to physical keys.
String get _predefinedHidCodeMap {
final OutputLines<int> lines = OutputLines<int>('Physical key map');
for (final PhysicalKeyEntry entry in keyData.entries) {
lines.add(entry.usbHidCode, ' ${toHex(entry.usbHidCode)}: ${entry.constantName},');
}
return lines.sortedJoin().trimRight();
}
/// This generates the map of Flutter key codes to logical keys.
String get _predefinedKeyCodeMap {
final OutputLines<int> lines = OutputLines<int>('Logical key map', behavior: DeduplicateBehavior.kSkip);
for (final LogicalKeyEntry entry in logicalData.entries) {
lines.add(entry.value, ' ${toHex(entry.value, digits: 11)}: ${entry.constantName},');
}
return lines.sortedJoin().trimRight();
}
String get _maskConstantVariables {
final OutputLines<int> lines = OutputLines<int>('Mask constants', behavior: DeduplicateBehavior.kKeep);
for (final MaskConstant constant in _maskConstants) {
lines.add(constant.value, '''
${_wrapString(constant.description)} ///
/// This is used by platform-specific code to generate Flutter key codes.
static const int ${constant.lowerCamelName} = ${toHex(constant.value, digits: 11)};
''');
}
return lines.join().trimRight();
}
@override
String get templatePath => path.join(dataRoot, 'keyboard_key.tmpl');
@override
Map<String, String> mappings() {
return <String, String>{
'LOGICAL_KEY_MAP': _predefinedKeyCodeMap,
'LOGICAL_KEY_DEFINITIONS': _logicalDefinitions,
'LOGICAL_KEY_SYNONYMS': _logicalSynonyms,
'LOGICAL_KEY_REVERSE_SYNONYMS': _logicalReverseSynonyms,
'LOGICAL_KEY_KEY_LABELS': _logicalKeyLabels,
'PHYSICAL_KEY_MAP': _predefinedHidCodeMap,
'PHYSICAL_KEY_DEFINITIONS': _physicalDefinitions,
'PHYSICAL_KEY_DEBUG_NAMES': _physicalDebugNames,
'MASK_CONSTANTS': _maskConstantVariables,
};
}
late final Map<String, SynonymKeyInfo> synonyms = Map<String, SynonymKeyInfo>.fromEntries(
LogicalKeyData.synonyms.entries.map((MapEntry<String, List<String>> synonymDefinition) {
final List<LogicalKeyEntry> entries = synonymDefinition.value.map(
(String name) => logicalData.entryByName(name)).toList();
return MapEntry<String, SynonymKeyInfo>(
synonymDefinition.key,
SynonymKeyInfo(
entries,
synonymDefinition.key,
),
);
}),
);
}
| flutter/dev/tools/gen_keycodes/lib/keyboard_keys_code_gen.dart/0 | {
"file_path": "flutter/dev/tools/gen_keycodes/lib/keyboard_keys_code_gen.dart",
"repo_id": "flutter",
"token_count": 2753
} | 545 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:convert';
import 'dart:io';
const String registry = 'https://www.iana.org/assignments/language-subtag-registry/language-subtag-registry';
/// A script to generate a Dart cache of https://www.iana.org. This should be
/// run occasionally. It was created since iana.org was found to be flaky.
///
/// To execute: dart gen_subtag_registry.dart > language_subtag_registry.dart
Future<void> main() async {
final HttpClient client = HttpClient();
final HttpClientRequest request = await client.getUrl(Uri.parse(registry));
final HttpClientResponse response = await request.close();
final String body = (await response.cast<List<int>>().transform<String>(utf8.decoder).toList()).join();
final File subtagRegistry = File('../language_subtag_registry.dart');
final File subtagRegistryFlutterTools = File('../../../../packages/flutter_tools/lib/src/localizations/language_subtag_registry.dart');
final String content = '''
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
/// Cache of $registry.
const String languageSubtagRegistry = \'\'\'$body\'\'\';''';
subtagRegistry.writeAsStringSync(content);
subtagRegistryFlutterTools.writeAsStringSync(content);
client.close(force: true);
}
| flutter/dev/tools/localization/bin/gen_subtag_registry.dart/0 | {
"file_path": "flutter/dev/tools/localization/bin/gen_subtag_registry.dart",
"repo_id": "flutter",
"token_count": 445
} | 546 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:io';
import 'dart:math';
import 'package:collection/collection.dart';
import 'package:meta/meta.dart';
import 'package:vector_math/vector_math_64.dart';
import 'package:xml/xml.dart';
// String to use for a single indentation.
const String kIndent = ' ';
/// Represents an animation, and provides logic to generate dart code for it.
class Animation {
const Animation(this.size, this.paths);
factory Animation.fromFrameData(List<FrameData> frames) {
_validateFramesData(frames);
final Point<double> size = frames[0].size;
final List<PathAnimation> paths = <PathAnimation>[];
for (int i = 0; i < frames[0].paths.length; i += 1) {
paths.add(PathAnimation.fromFrameData(frames, i));
}
return Animation(size, paths);
}
/// The size of the animation (width, height) in pixels.
final Point<double> size;
/// List of paths in the animation.
final List<PathAnimation> paths;
static void _validateFramesData(List<FrameData> frames) {
final Point<double> size = frames[0].size;
final int numPaths = frames[0].paths.length;
for (int i = 0; i < frames.length; i += 1) {
final FrameData frame = frames[i];
if (size != frame.size) {
throw Exception(
'All animation frames must have the same size,\n'
'first frame size was: (${size.x}, ${size.y})\n'
'frame $i size was: (${frame.size.x}, ${frame.size.y})'
);
}
if (numPaths != frame.paths.length) {
throw Exception(
'All animation frames must have the same number of paths,\n'
'first frame has $numPaths paths\n'
'frame $i has ${frame.paths.length} paths'
);
}
}
}
String toDart(String className, String varName) {
final StringBuffer sb = StringBuffer();
sb.write('const $className $varName = const $className(\n');
sb.write('${kIndent}const Size(${size.x}, ${size.y}),\n');
sb.write('${kIndent}const <_PathFrames>[\n');
for (final PathAnimation path in paths) {
sb.write(path.toDart());
}
sb.write('$kIndent],\n');
sb.write(');');
return sb.toString();
}
}
/// Represents the animation of a single path.
class PathAnimation {
const PathAnimation(this.commands, {required this.opacities});
factory PathAnimation.fromFrameData(List<FrameData> frames, int pathIdx) {
if (frames.isEmpty) {
return const PathAnimation(<PathCommandAnimation>[], opacities: <double>[]);
}
final List<PathCommandAnimation> commands = <PathCommandAnimation>[];
for (int commandIdx = 0; commandIdx < frames[0].paths[pathIdx].commands.length; commandIdx += 1) {
final int numPointsInCommand = frames[0].paths[pathIdx].commands[commandIdx].points.length;
final List<List<Point<double>>> points = List<List<Point<double>>>.filled(numPointsInCommand, <Point<double>>[]);
final String commandType = frames[0].paths[pathIdx].commands[commandIdx].type;
for (int i = 0; i < frames.length; i += 1) {
final FrameData frame = frames[i];
final String currentCommandType = frame.paths[pathIdx].commands[commandIdx].type;
if (commandType != currentCommandType) {
throw Exception(
'Paths must be built from the same commands in all frames '
"command $commandIdx at frame 0 was of type '$commandType' "
"command $commandIdx at frame $i was of type '$currentCommandType'"
);
}
for (int j = 0; j < numPointsInCommand; j += 1) {
points[j].add(frame.paths[pathIdx].commands[commandIdx].points[j]);
}
}
commands.add(PathCommandAnimation(commandType, points));
}
final List<double> opacities =
frames.map<double>((FrameData d) => d.paths[pathIdx].opacity).toList();
return PathAnimation(commands, opacities: opacities);
}
/// List of commands for drawing the path.
final List<PathCommandAnimation> commands;
/// The path opacity for each animation frame.
final List<double> opacities;
@override
String toString() {
return 'PathAnimation(commands: $commands, opacities: $opacities)';
}
String toDart() {
final StringBuffer sb = StringBuffer();
sb.write('${kIndent * 2}const _PathFrames(\n');
sb.write('${kIndent * 3}opacities: const <double>[\n');
for (final double opacity in opacities) {
sb.write('${kIndent * 4}$opacity,\n');
}
sb.write('${kIndent * 3}],\n');
sb.write('${kIndent * 3}commands: const <_PathCommand>[\n');
for (final PathCommandAnimation command in commands) {
sb.write(command.toDart());
}
sb.write('${kIndent * 3}],\n');
sb.write('${kIndent * 2}),\n');
return sb.toString();
}
}
/// Represents the animation of a single path command.
class PathCommandAnimation {
const PathCommandAnimation(this.type, this.points);
/// The command type.
final String type;
/// A matrix with the command's points in different frames.
///
/// points[i][j] is the i-th point of the command at frame j.
final List<List<Point<double>>> points;
@override
String toString() {
return 'PathCommandAnimation(type: $type, points: $points)';
}
String toDart() {
final String dartCommandClass = switch (type) {
'M' => '_PathMoveTo',
'C' => '_PathCubicTo',
'L' => '_PathLineTo',
'Z' => '_PathClose',
_ => throw Exception('unsupported path command: $type'),
};
final StringBuffer sb = StringBuffer();
sb.write('${kIndent * 4}const $dartCommandClass(\n');
for (final List<Point<double>> pointFrames in points) {
sb.write('${kIndent * 5}const <Offset>[\n');
for (final Point<double> point in pointFrames) {
sb.write('${kIndent * 6}const Offset(${point.x}, ${point.y}),\n');
}
sb.write('${kIndent * 5}],\n');
}
sb.write('${kIndent * 4}),\n');
return sb.toString();
}
}
/// Interprets some subset of an SVG file.
///
/// Recursively goes over the SVG tree, applying transforms and opacities,
/// and build a FrameData which is a flat representation of the paths in the SVG
/// file, after applying transformations and converting relative coordinates to
/// absolute.
///
/// This does not support the SVG specification, but is just built to
/// support SVG files exported by a specific tool the motion design team is
/// using.
FrameData interpretSvg(String svgFilePath) {
final File file = File(svgFilePath);
final String fileData = file.readAsStringSync();
final XmlElement svgElement = _extractSvgElement(XmlDocument.parse(fileData));
final double width = parsePixels(_extractAttr(svgElement, 'width')).toDouble();
final double height = parsePixels(_extractAttr(svgElement, 'height')).toDouble();
final List<SvgPath> paths =
_interpretSvgGroup(svgElement.children, _Transform());
return FrameData(Point<double>(width, height), paths);
}
List<SvgPath> _interpretSvgGroup(List<XmlNode> children, _Transform transform) {
final List<SvgPath> paths = <SvgPath>[];
for (final XmlNode node in children) {
if (node.nodeType != XmlNodeType.ELEMENT) {
continue;
}
final XmlElement element = node as XmlElement;
if (element.name.local == 'path') {
paths.add(SvgPath.fromElement(element)._applyTransform(transform));
}
if (element.name.local == 'g') {
double opacity = transform.opacity;
if (_hasAttr(element, 'opacity')) {
opacity *= double.parse(_extractAttr(element, 'opacity'));
}
Matrix3 transformMatrix = transform.transformMatrix;
if (_hasAttr(element, 'transform')) {
transformMatrix = transformMatrix.multiplied(
_parseSvgTransform(_extractAttr(element, 'transform')));
}
final _Transform subtreeTransform = _Transform(
transformMatrix: transformMatrix,
opacity: opacity,
);
paths.addAll(_interpretSvgGroup(element.children, subtreeTransform));
}
}
return paths;
}
// Given a points list in the form e.g: "25.0, 1.0 12.0, 12.0 23.0, 9.0" matches
// the coordinated of the first point and the rest of the string, for the
// example above:
// group 1 will match "25.0"
// group 2 will match "1.0"
// group 3 will match "12.0, 12.0 23.0, 9.0"
//
// Commas are optional.
final RegExp _pointMatcher = RegExp(r'^ *([\-\.0-9]+) *,? *([\-\.0-9]+)(.*)');
/// Parse a string with a list of points, e.g:
/// '25.0, 1.0 12.0, 12.0 23.0, 9.0' will be parsed to:
/// [Point(25.0, 1.0), Point(12.0, 12.0), Point(23.0, 9.0)].
///
/// Commas are optional.
List<Point<double>> parsePoints(String points) {
String unParsed = points;
final List<Point<double>> result = <Point<double>>[];
while (unParsed.isNotEmpty && _pointMatcher.hasMatch(unParsed)) {
final Match m = _pointMatcher.firstMatch(unParsed)!;
result.add(Point<double>(
double.parse(m.group(1)!),
double.parse(m.group(2)!),
));
unParsed = m.group(3)!;
}
return result;
}
/// Data for a single animation frame.
@immutable
class FrameData {
const FrameData(this.size, this.paths);
final Point<double> size;
final List<SvgPath> paths;
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is FrameData
&& other.size == size
&& const ListEquality<SvgPath>().equals(other.paths, paths);
}
@override
int get hashCode => Object.hash(size, Object.hashAll(paths));
@override
String toString() {
return 'FrameData(size: $size, paths: $paths)';
}
}
/// Represents an SVG path element.
@immutable
class SvgPath {
const SvgPath(this.id, this.commands, {this.opacity = 1.0});
final String id;
final List<SvgPathCommand> commands;
final double opacity;
static const String _pathCommandAtom = r' *([a-zA-Z]) *([\-\.0-9 ,]*)';
static final RegExp _pathCommandValidator = RegExp('^($_pathCommandAtom)*\$');
static final RegExp _pathCommandMatcher = RegExp(_pathCommandAtom);
static SvgPath fromElement(XmlElement pathElement) {
assert(pathElement.name.local == 'path');
final String id = _extractAttr(pathElement, 'id');
final String dAttr = _extractAttr(pathElement, 'd');
final List<SvgPathCommand> commands = <SvgPathCommand>[];
final SvgPathCommandBuilder commandsBuilder = SvgPathCommandBuilder();
if (!_pathCommandValidator.hasMatch(dAttr)) {
throw Exception('illegal or unsupported path d expression: $dAttr');
}
for (final Match match in _pathCommandMatcher.allMatches(dAttr)) {
final String commandType = match.group(1)!;
final String pointStr = match.group(2)!;
commands.add(commandsBuilder.build(commandType, parsePoints(pointStr)));
}
return SvgPath(id, commands);
}
SvgPath _applyTransform(_Transform transform) {
final List<SvgPathCommand> transformedCommands =
commands.map<SvgPathCommand>((SvgPathCommand c) => c._applyTransform(transform)).toList();
return SvgPath(id, transformedCommands, opacity: opacity * transform.opacity);
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is SvgPath
&& other.id == id
&& other.opacity == opacity
&& const ListEquality<SvgPathCommand>().equals(other.commands, commands);
}
@override
int get hashCode => Object.hash(id, Object.hashAll(commands), opacity);
@override
String toString() {
return 'SvgPath(id: $id, opacity: $opacity, commands: $commands)';
}
}
/// Represents a single SVG path command from an SVG d element.
///
/// This class normalizes all the 'd' commands into a single type, that has
/// a command type and a list of points.
///
/// Some examples of how d commands translated to SvgPathCommand:
/// * "M 0.0, 1.0" => SvgPathCommand('M', [Point(0.0, 1.0)])
/// * "Z" => SvgPathCommand('Z', [])
/// * "C 1.0, 1.0 2.0, 2.0 3.0, 3.0" SvgPathCommand('C', [Point(1.0, 1.0),
/// Point(2.0, 2.0), Point(3.0, 3.0)])
@immutable
class SvgPathCommand {
const SvgPathCommand(this.type, this.points);
/// The command type.
final String type;
/// List of points used by this command.
final List<Point<double>> points;
SvgPathCommand _applyTransform(_Transform transform) {
final List<Point<double>> transformedPoints =
_vector3ArrayToPoints(
transform.transformMatrix.applyToVector3Array(
_pointsToVector3Array(points)
)
);
return SvgPathCommand(type, transformedPoints);
}
@override
bool operator ==(Object other) {
if (other.runtimeType != runtimeType) {
return false;
}
return other is SvgPathCommand
&& other.type == type
&& const ListEquality<Point<double>>().equals(other.points, points);
}
@override
int get hashCode => Object.hash(type, Object.hashAll(points));
@override
String toString() {
return 'SvgPathCommand(type: $type, points: $points)';
}
}
class SvgPathCommandBuilder {
static const Map<String, void> kRelativeCommands = <String, void> {
'c': null,
'l': null,
'm': null,
't': null,
's': null,
};
Point<double> lastPoint = const Point<double>(0.0, 0.0);
Point<double> subPathStartPoint = const Point<double>(0.0, 0.0);
SvgPathCommand build(String type, List<Point<double>> points) {
List<Point<double>> absPoints = points;
if (_isRelativeCommand(type)) {
absPoints = points.map<Point<double>>((Point<double> p) => p + lastPoint).toList();
}
if (type == 'M' || type == 'm') {
subPathStartPoint = absPoints.last;
}
if (type == 'Z' || type == 'z') {
lastPoint = subPathStartPoint;
} else {
lastPoint = absPoints.last;
}
return SvgPathCommand(type.toUpperCase(), absPoints);
}
static bool _isRelativeCommand(String type) {
return kRelativeCommands.containsKey(type);
}
}
List<double> _pointsToVector3Array(List<Point<double>> points) {
final List<double> result = List<double>.filled(points.length * 3, 0.0);
for (int i = 0; i < points.length; i += 1) {
result[i * 3] = points[i].x;
result[i * 3 + 1] = points[i].y;
result[i * 3 + 2] = 1.0;
}
return result;
}
List<Point<double>> _vector3ArrayToPoints(List<double> vector) {
final int numPoints = (vector.length / 3).floor();
final List<Point<double>> points = <Point<double>>[
for (int i = 0; i < numPoints; i += 1)
Point<double>(vector[i*3], vector[i*3 + 1]),
];
return points;
}
/// Represents a transformation to apply on an SVG subtree.
///
/// This includes more transforms than the ones described by the SVG transform
/// attribute, e.g opacity.
class _Transform {
/// Constructs a new _Transform, default arguments create a no-op transform.
_Transform({Matrix3? transformMatrix, this.opacity = 1.0}) :
transformMatrix = transformMatrix ?? Matrix3.identity();
final Matrix3 transformMatrix;
final double opacity;
_Transform applyTransform(_Transform transform) {
return _Transform(
transformMatrix: transform.transformMatrix.multiplied(transformMatrix),
opacity: transform.opacity * opacity,
);
}
}
const String _transformCommandAtom = r' *([^(]+)\(([^)]*)\)';
final RegExp _transformValidator = RegExp('^($_transformCommandAtom)*\$');
final RegExp _transformCommand = RegExp(_transformCommandAtom);
Matrix3 _parseSvgTransform(String transform) {
if (!_transformValidator.hasMatch(transform)) {
throw Exception('illegal or unsupported transform: $transform');
}
final Iterable<Match> matches =_transformCommand.allMatches(transform).toList().reversed;
Matrix3 result = Matrix3.identity();
for (final Match m in matches) {
final String command = m.group(1)!;
final String params = m.group(2)!;
if (command == 'translate') {
result = _parseSvgTranslate(params).multiplied(result);
continue;
}
if (command == 'scale') {
result = _parseSvgScale(params).multiplied(result);
continue;
}
if (command == 'rotate') {
result = _parseSvgRotate(params).multiplied(result);
continue;
}
throw Exception('unimplemented transform: $command');
}
return result;
}
final RegExp _valueSeparator = RegExp('( *, *| +)');
Matrix3 _parseSvgTranslate(String paramsStr) {
final List<String> params = paramsStr.split(_valueSeparator);
assert(params.isNotEmpty);
assert(params.length <= 2);
final double x = double.parse(params[0]);
final double y = params.length < 2 ? 0 : double.parse(params[1]);
return _matrix(1.0, 0.0, 0.0, 1.0, x, y);
}
Matrix3 _parseSvgScale(String paramsStr) {
final List<String> params = paramsStr.split(_valueSeparator);
assert(params.isNotEmpty);
assert(params.length <= 2);
final double x = double.parse(params[0]);
final double y = params.length < 2 ? 0 : double.parse(params[1]);
return _matrix(x, 0.0, 0.0, y, 0.0, 0.0);
}
Matrix3 _parseSvgRotate(String paramsStr) {
final List<String> params = paramsStr.split(_valueSeparator);
assert(params.length == 1);
final double a = radians(double.parse(params[0]));
return _matrix(cos(a), sin(a), -sin(a), cos(a), 0.0, 0.0);
}
Matrix3 _matrix(double a, double b, double c, double d, double e, double f) {
return Matrix3(a, b, 0.0, c, d, 0.0, e, f, 1.0);
}
// Matches a pixels expression e.g "14px".
// First group is just the number.
final RegExp _pixelsExp = RegExp(r'^([0-9]+)px$');
/// Parses a pixel expression, e.g "14px", and returns the number.
/// Throws an [ArgumentError] if the given string doesn't match the pattern.
int parsePixels(String pixels) {
if (!_pixelsExp.hasMatch(pixels)) {
throw ArgumentError(
"illegal pixels expression: '$pixels'"
' (the tool currently only support pixel units).');
}
return int.parse(_pixelsExp.firstMatch(pixels)!.group(1)!);
}
String _extractAttr(XmlElement element, String name) {
try {
return element.attributes.singleWhere((XmlAttribute x) => x.name.local == name)
.value;
} catch (e) {
throw ArgumentError(
"Can't find a single '$name' attributes in ${element.name}, "
'attributes were: ${element.attributes}'
);
}
}
bool _hasAttr(XmlElement element, String name) {
return element.attributes.where((XmlAttribute a) => a.name.local == name).isNotEmpty;
}
XmlElement _extractSvgElement(XmlDocument document) {
return document.children.singleWhere(
(XmlNode node) => node.nodeType == XmlNodeType.ELEMENT &&
_asElement(node).name.local == 'svg'
) as XmlElement;
}
XmlElement _asElement(XmlNode node) => node as XmlElement;
| flutter/dev/tools/vitool/lib/vitool.dart/0 | {
"file_path": "flutter/dev/tools/vitool/lib/vitool.dart",
"repo_id": "flutter",
"token_count": 6919
} | 547 |
<svg id="svg_build_00" xmlns="http://www.w3.org/2000/svg" width="48px" height="48px" >
<path id="path_1" d=" z" fill="#000000" />
</svg>
| flutter/dev/tools/vitool/test_assets/leading_space_path_command.svg/0 | {
"file_path": "flutter/dev/tools/vitool/test_assets/leading_space_path_command.svg",
"repo_id": "flutter",
"token_count": 64
} | 548 |
Flutter Examples
================
This directory contains several examples of using Flutter. To run an example,
use `flutter run` inside that example's directory. See the [getting started
guide](https://flutter.dev/getting-started/) to install the `flutter` tool.
For additional samples, see the
[`flutter/samples`](https://github.com/flutter/samples) repo.
Available examples include:
- **Hello, world** The [hello world app](hello_world) is a minimal Flutter app
that shows the text "Hello, world!"
- **Flutter gallery** The flutter gallery app no longer lives in this repo.
Please see the [gallery repo](https://github.com/flutter/gallery).
- **Layers** The [layers vignettes](layers) show how to use the various layers
in the Flutter framework. For details, see the [layers
README](layers/README.md).
- **Platform Channel** The [platform channel app](platform_channel) demonstrates
how to connect a Flutter app to platform-specific APIs. For documentation, see
<https://flutter.dev/platform-channels/>.
- **Platform Channel Swift** The [platform channel swift
app](platform_channel_swift) is the same as [platform
channel](platform_channel) but the iOS version is in Swift and there is no
Android version.
## Notes
Note on Gradle wrapper files in `.gitignore`:
Gradle wrapper files should normally be checked into source control. The example
projects don't do that to avoid having several copies of the wrapper binary in
the Flutter repo. Instead, the Gradle wrapper is injected by Flutter tooling,
and the wrapper files are .gitignore'd to avoid making the Flutter repository
dirty as a side effect of running the examples.
| flutter/examples/README.md/0 | {
"file_path": "flutter/examples/README.md",
"repo_id": "flutter",
"token_count": 437
} | 549 |
// Copyright 2014 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
/// Flutter code sample for [CupertinoContextMenu].
void main() => runApp(const ContextMenuApp());
class ContextMenuApp extends StatelessWidget {
const ContextMenuApp({super.key});
@override
Widget build(BuildContext context) {
return const CupertinoApp(
theme: CupertinoThemeData(brightness: Brightness.light),
home: ContextMenuExample(),
);
}
}
class ContextMenuExample extends StatelessWidget {
const ContextMenuExample({super.key});
@override
Widget build(BuildContext context) {
return CupertinoPageScaffold(
navigationBar: const CupertinoNavigationBar(
middle: Text('CupertinoContextMenu Sample'),
),
child: Center(
child: SizedBox(
width: 100,
height: 100,
child: CupertinoContextMenu(
actions: <Widget>[
CupertinoContextMenuAction(
onPressed: () {
Navigator.pop(context);
},
isDefaultAction: true,
trailingIcon: CupertinoIcons.doc_on_clipboard_fill,
child: const Text('Copy'),
),
CupertinoContextMenuAction(
onPressed: () {
Navigator.pop(context);
},
trailingIcon: CupertinoIcons.share,
child: const Text('Share'),
),
CupertinoContextMenuAction(
onPressed: () {
Navigator.pop(context);
},
trailingIcon: CupertinoIcons.heart,
child: const Text('Favorite'),
),
CupertinoContextMenuAction(
onPressed: () {
Navigator.pop(context);
},
isDestructiveAction: true,
trailingIcon: CupertinoIcons.delete,
child: const Text('Delete'),
),
],
child: const ColoredBox(
color: CupertinoColors.systemYellow,
child: FlutterLogo(size: 500.0),
),
),
),
),
);
}
}
| flutter/examples/api/lib/cupertino/context_menu/cupertino_context_menu.0.dart/0 | {
"file_path": "flutter/examples/api/lib/cupertino/context_menu/cupertino_context_menu.0.dart",
"repo_id": "flutter",
"token_count": 1160
} | 550 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.