text
stringlengths 6
13.6M
| id
stringlengths 13
176
| metadata
dict | __index_level_0__
int64 0
1.69k
|
---|---|---|---|
// ignore_for_file: prefer_const_constructors
import 'package:news_blocks/news_blocks.dart';
import 'package:test/test.dart';
void main() {
group('ImageBlock', () {
test('can be (de)serialized', () {
final block = ImageBlock(imageUrl: 'imageUrl');
expect(ImageBlock.fromJson(block.toJson()), equals(block));
});
});
}
| news_toolkit/flutter_news_example/api/packages/news_blocks/test/src/image_block_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/api/packages/news_blocks/test/src/image_block_test.dart",
"repo_id": "news_toolkit",
"token_count": 129
} | 851 |
// ignore_for_file: prefer_const_constructors
import 'package:news_blocks/news_blocks.dart';
import 'package:test/test.dart';
void main() {
group('TextHeadlineBlock', () {
test('can be (de)serialized', () {
final block = TextHeadlineBlock(text: 'Title');
expect(TextHeadlineBlock.fromJson(block.toJson()), equals(block));
});
});
}
| news_toolkit/flutter_news_example/api/packages/news_blocks/test/src/text_headline_block_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/api/packages/news_blocks/test/src/text_headline_block_test.dart",
"repo_id": "news_toolkit",
"token_count": 133
} | 852 |
import 'dart:io';
import 'package:dart_frog/dart_frog.dart';
import 'package:flutter_news_example_api/api.dart';
import 'package:news_blocks/news_blocks.dart';
Future<Response> onRequest(RequestContext context) async {
if (context.request.method != HttpMethod.get) {
return Response(statusCode: HttpStatus.methodNotAllowed);
}
final term = context.request.url.queryParameters['q'];
if (term == null) return Response(statusCode: HttpStatus.badRequest);
final newsDataSource = context.read<NewsDataSource>();
final results = await Future.wait([
newsDataSource.getRelevantArticles(term: term),
newsDataSource.getRelevantTopics(term: term),
]);
final articles = results.first as List<NewsBlock>;
final topics = results.last as List<String>;
final response = RelevantSearchResponse(articles: articles, topics: topics);
return Response.json(body: response);
}
| news_toolkit/flutter_news_example/api/routes/api/v1/search/relevant.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/api/routes/api/v1/search/relevant.dart",
"repo_id": "news_toolkit",
"token_count": 279
} | 853 |
// ignore_for_file: prefer_const_constructors
import 'package:flutter_news_example_api/api.dart';
import 'package:news_blocks/news_blocks.dart';
import 'package:test/test.dart';
void main() {
group('Article', () {
test('can be (de)serialized', () {
final blockA = SectionHeaderBlock(title: 'sectionA');
final blockB = SectionHeaderBlock(title: 'sectionB');
final article = Article(
title: 'title',
blocks: [blockA, blockB],
totalBlocks: 2,
url: Uri.parse('http://flutter.dev'),
);
expect(Article.fromJson(article.toJson()), equals(article));
});
});
}
| news_toolkit/flutter_news_example/api/test/src/data/models/article_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/api/test/src/data/models/article_test.dart",
"repo_id": "news_toolkit",
"token_count": 249
} | 854 |
part of 'analytics_bloc.dart';
abstract class AnalyticsState extends Equatable {
const AnalyticsState();
}
class AnalyticsInitial extends AnalyticsState {
@override
List<Object> get props => [];
}
| news_toolkit/flutter_news_example/lib/analytics/bloc/analytics_state.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/lib/analytics/bloc/analytics_state.dart",
"repo_id": "news_toolkit",
"token_count": 58
} | 855 |
import 'package:app_ui/app_ui.dart';
import 'package:flutter/material.dart' hide Spacer;
import 'package:flutter_bloc/flutter_bloc.dart';
import 'package:flutter_news_example/article/article.dart';
import 'package:flutter_news_example/l10n/l10n.dart';
class ArticleComments extends StatelessWidget {
const ArticleComments({super.key});
@override
Widget build(BuildContext context) {
return Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
context.l10n.discussion,
style: Theme.of(context).textTheme.displaySmall,
key: const Key('articleComments_discussionTitle'),
),
const SizedBox(height: AppSpacing.lg),
ConstrainedBox(
constraints: const BoxConstraints(
maxHeight: 148,
),
child: AppTextField(
hintText: context.l10n.commentEntryHint,
onSubmitted: (_) {
final articleBloc = context.read<ArticleBloc>();
articleBloc.add(
ArticleCommented(articleTitle: articleBloc.state.title!),
);
},
),
),
],
);
}
}
| news_toolkit/flutter_news_example/lib/article/widgets/article_comments.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/lib/article/widgets/article_comments.dart",
"repo_id": "news_toolkit",
"token_count": 537
} | 856 |
part of 'feed_bloc.dart';
abstract class FeedEvent extends Equatable {
const FeedEvent();
}
class FeedRequested extends FeedEvent {
const FeedRequested({
required this.category,
});
final Category category;
@override
List<Object> get props => [category];
}
class FeedRefreshRequested extends FeedEvent {
const FeedRefreshRequested({required this.category});
final Category category;
@override
List<Object> get props => [category];
}
class FeedResumed extends FeedEvent {
const FeedResumed();
@override
List<Object> get props => [];
}
| news_toolkit/flutter_news_example/lib/feed/bloc/feed_event.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/lib/feed/bloc/feed_event.dart",
"repo_id": "news_toolkit",
"token_count": 170
} | 857 |
import 'package:flutter_gen/gen_l10n/app_localizations.dart';
import 'package:flutter/widgets.dart';
export 'package:flutter_gen/gen_l10n/app_localizations.dart';
extension AppLocalizationsX on BuildContext {
AppLocalizations get l10n => AppLocalizations.of(this);
}
| news_toolkit/flutter_news_example/lib/l10n/l10n.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/lib/l10n/l10n.dart",
"repo_id": "news_toolkit",
"token_count": 95
} | 858 |
import 'package:app_ui/app_ui.dart'
show AppButton, AppColors, AppSpacing, Assets;
import 'package:email_launcher/email_launcher.dart';
import 'package:flutter/material.dart';
import 'package:flutter_news_example/l10n/l10n.dart';
class MagicLinkPromptView extends StatelessWidget {
const MagicLinkPromptView({required this.email, super.key});
final String email;
@override
Widget build(BuildContext context) {
return CustomScrollView(
slivers: [
SliverFillRemaining(
hasScrollBody: false,
child: Padding(
padding: const EdgeInsets.fromLTRB(
AppSpacing.xlg,
AppSpacing.xlg,
AppSpacing.xlg,
AppSpacing.xxlg,
),
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
const MagicLinkPromptHeader(),
const SizedBox(height: AppSpacing.xxxlg),
Assets.icons.envelopeOpen.svg(),
const SizedBox(height: AppSpacing.xxxlg),
MagicLinkPromptSubtitle(email: email),
const Spacer(),
MagicLinkPromptOpenEmailButton()
],
),
),
),
],
);
}
}
@visibleForTesting
class MagicLinkPromptHeader extends StatelessWidget {
const MagicLinkPromptHeader({super.key});
@override
Widget build(BuildContext context) {
return Text(
context.l10n.magicLinkPromptHeader,
style: Theme.of(context).textTheme.displaySmall,
);
}
}
@visibleForTesting
class MagicLinkPromptSubtitle extends StatelessWidget {
const MagicLinkPromptSubtitle({required this.email, super.key});
final String email;
@override
Widget build(BuildContext context) {
final theme = Theme.of(context);
return Column(
children: [
Text(
context.l10n.magicLinkPromptTitle,
textAlign: TextAlign.center,
style: theme.textTheme.bodyLarge,
),
Text(
email,
textAlign: TextAlign.center,
style: theme.textTheme.bodyLarge?.apply(
color: AppColors.darkAqua,
),
),
const SizedBox(height: AppSpacing.xxlg),
Text(
context.l10n.magicLinkPromptSubtitle,
textAlign: TextAlign.center,
style: theme.textTheme.bodyLarge,
),
],
);
}
}
@visibleForTesting
class MagicLinkPromptOpenEmailButton extends StatelessWidget {
MagicLinkPromptOpenEmailButton({
EmailLauncher? emailLauncher,
super.key,
}) : _emailLauncher = emailLauncher ?? EmailLauncher();
final EmailLauncher _emailLauncher;
@override
Widget build(BuildContext context) {
return AppButton.darkAqua(
key: const Key('magicLinkPrompt_openMailButton_appButton'),
onPressed: _emailLauncher.launchEmailApp,
child: Text(context.l10n.openMailAppButtonText),
);
}
}
| news_toolkit/flutter_news_example/lib/magic_link_prompt/view/magic_link_prompt_view.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/lib/magic_link_prompt/view/magic_link_prompt_view.dart",
"repo_id": "news_toolkit",
"token_count": 1319
} | 859 |
import 'dart:async';
import 'package:bloc/bloc.dart';
import 'package:equatable/equatable.dart';
import 'package:form_inputs/form_inputs.dart';
import 'package:news_repository/news_repository.dart';
part 'newsletter_event.dart';
part 'newsletter_state.dart';
class NewsletterBloc extends Bloc<NewsletterEvent, NewsletterState> {
NewsletterBloc({
required this.newsRepository,
}) : super(const NewsletterState()) {
on<NewsletterSubscribed>(_onNewsletterSubscribed);
on<EmailChanged>(_onEmailChanged);
}
final NewsRepository newsRepository;
Future<void> _onNewsletterSubscribed(
NewsletterSubscribed event,
Emitter<NewsletterState> emit,
) async {
if (state.email.value.isEmpty) return;
emit(state.copyWith(status: NewsletterStatus.loading));
try {
await newsRepository.subscribeToNewsletter(email: state.email.value);
emit(state.copyWith(status: NewsletterStatus.success));
} catch (error, stackTrace) {
emit(state.copyWith(status: NewsletterStatus.failure));
addError(error, stackTrace);
}
}
Future<void> _onEmailChanged(
EmailChanged event,
Emitter<NewsletterState> emit,
) async {
final email = Email.dirty(event.email);
emit(
state.copyWith(
email: email,
isValid: Formz.validate([email]),
),
);
}
}
| news_toolkit/flutter_news_example/lib/newsletter/bloc/newsletter_bloc.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/lib/newsletter/bloc/newsletter_bloc.dart",
"repo_id": "news_toolkit",
"token_count": 484
} | 860 |
export 'bloc/onboarding_bloc.dart';
export 'view/view.dart';
export 'widgets/widgets.dart';
| news_toolkit/flutter_news_example/lib/onboarding/onboarding.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/lib/onboarding/onboarding.dart",
"repo_id": "news_toolkit",
"token_count": 37
} | 861 |
export 'view/view.dart';
| news_toolkit/flutter_news_example/lib/slideshow/slideshow.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/lib/slideshow/slideshow.dart",
"repo_id": "news_toolkit",
"token_count": 10
} | 862 |
export 'subscribe_modal.dart';
export 'subscribe_with_article_limit_modal.dart';
export 'subscription_card.dart';
| news_toolkit/flutter_news_example/lib/subscriptions/widgets/widgets.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/lib/subscriptions/widgets/widgets.dart",
"repo_id": "news_toolkit",
"token_count": 41
} | 863 |
import 'package:app_ui/app_ui.dart'
show AppBackButton, AppButton, AppColors, AppSpacing, AppSwitch, Assets;
import 'package:flutter/material.dart';
import 'package:flutter_bloc/flutter_bloc.dart';
import 'package:flutter_news_example/analytics/analytics.dart';
import 'package:flutter_news_example/app/app.dart';
import 'package:flutter_news_example/l10n/l10n.dart';
import 'package:flutter_news_example/notification_preferences/notification_preferences.dart';
import 'package:flutter_news_example/subscriptions/subscriptions.dart';
import 'package:flutter_news_example/terms_of_service/terms_of_service.dart';
import 'package:flutter_news_example/user_profile/user_profile.dart';
import 'package:notifications_repository/notifications_repository.dart';
import 'package:user_repository/user_repository.dart';
class UserProfilePage extends StatelessWidget {
const UserProfilePage({super.key});
static MaterialPageRoute<void> route() {
return MaterialPageRoute(builder: (_) => const UserProfilePage());
}
@override
Widget build(BuildContext context) {
return BlocProvider(
create: (_) => UserProfileBloc(
userRepository: context.read<UserRepository>(),
notificationsRepository: context.read<NotificationsRepository>(),
),
child: const UserProfileView(),
);
}
}
@visibleForTesting
class UserProfileView extends StatefulWidget {
const UserProfileView({super.key});
@override
State<UserProfileView> createState() => _UserProfileViewState();
}
class _UserProfileViewState extends State<UserProfileView>
with WidgetsBindingObserver {
@override
void initState() {
super.initState();
WidgetsBinding.instance.addObserver(this);
context.read<UserProfileBloc>().add(const FetchNotificationsEnabled());
}
@override
void didChangeAppLifecycleState(AppLifecycleState state) {
// Fetch current notification status each time a user enters the app.
// This may happen when a user changes permissions in app settings.
if (state == AppLifecycleState.resumed) {
WidgetsFlutterBinding.ensureInitialized();
context.read<UserProfileBloc>().add(const FetchNotificationsEnabled());
}
}
@override
void dispose() {
WidgetsBinding.instance.removeObserver(this);
super.dispose();
}
@override
Widget build(BuildContext context) {
final user = context.select((UserProfileBloc bloc) => bloc.state.user);
final notificationsEnabled = context
.select((UserProfileBloc bloc) => bloc.state.notificationsEnabled);
final isUserSubscribed = context.select<AppBloc, bool>(
(bloc) => bloc.state.isUserSubscribed,
);
final l10n = context.l10n;
return BlocListener<UserProfileBloc, UserProfileState>(
listener: (context, state) {
if (state.status == UserProfileStatus.togglingNotificationsSucceeded &&
state.notificationsEnabled) {
context
.read<AnalyticsBloc>()
.add(TrackAnalyticsEvent(PushNotificationSubscriptionEvent()));
}
},
child: BlocListener<AppBloc, AppState>(
listener: (context, state) {
if (state.status == AppStatus.unauthenticated) {
Navigator.of(context).pop();
}
},
child: Scaffold(
appBar: AppBar(
leading: const AppBackButton(),
),
body: CustomScrollView(
slivers: [
SliverToBoxAdapter(
child: Column(
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
const UserProfileTitle(),
if (!user.isAnonymous) ...[
UserProfileItem(
key: const Key('userProfilePage_userItem'),
leading: Assets.icons.profileIcon.svg(),
title: user.email ?? '',
),
const UserProfileLogoutButton(),
],
const SizedBox(height: AppSpacing.lg),
const _UserProfileDivider(),
UserProfileSubtitle(
subtitle: l10n.userProfileSubscriptionDetailsSubtitle,
),
if (isUserSubscribed)
UserProfileItem(
key: const Key('userProfilePage_subscriptionItem'),
title: l10n.manageSubscriptionTile,
trailing: const Icon(Icons.chevron_right),
onTap: () => Navigator.of(context).push(
ManageSubscriptionPage.route(),
),
)
else
UserProfileSubscribeBox(
onSubscribePressed: () =>
showPurchaseSubscriptionDialog(context: context),
),
const _UserProfileDivider(),
UserProfileSubtitle(
subtitle: l10n.userProfileSettingsSubtitle,
),
UserProfileItem(
key: const Key('userProfilePage_notificationsItem'),
leading: Assets.icons.notificationsIcon.svg(),
title: l10n.userProfileSettingsNotificationsTitle,
trailing: AppSwitch(
onText: l10n.checkboxOnTitle,
offText: l10n.userProfileCheckboxOffTitle,
value: notificationsEnabled,
onChanged: (_) => context
.read<UserProfileBloc>()
.add(const ToggleNotifications()),
),
),
UserProfileItem(
key: const Key(
'userProfilePage_notificationPreferencesItem',
),
title: l10n.notificationPreferencesTitle,
trailing: const Icon(
Icons.chevron_right,
key: Key(
'''userProfilePage_notificationPreferencesItem_trailing''',
),
),
onTap: () => Navigator.of(context).push(
NotificationPreferencesPage.route(),
),
),
const _UserProfileDivider(),
UserProfileSubtitle(
subtitle: l10n.userProfileLegalSubtitle,
),
UserProfileItem(
key: const Key('userProfilePage_termsOfServiceItem'),
leading: Assets.icons.termsOfUseIcon.svg(),
title:
l10n.userProfileLegalTermsOfUseAndPrivacyPolicyTitle,
onTap: () => Navigator.of(context)
.push<void>(TermsOfServicePage.route()),
),
UserProfileItem(
key: const Key('userProfilePage_aboutItem'),
leading: Assets.icons.aboutIcon.svg(),
title: l10n.userProfileLegalAboutTitle,
),
],
),
),
],
),
),
),
);
}
}
@visibleForTesting
class UserProfileTitle extends StatelessWidget {
const UserProfileTitle({super.key});
@override
Widget build(BuildContext context) {
final theme = Theme.of(context);
return Padding(
padding: const EdgeInsets.all(AppSpacing.lg),
child: Text(
context.l10n.userProfileTitle,
style: theme.textTheme.displaySmall,
),
);
}
}
@visibleForTesting
class UserProfileSubtitle extends StatelessWidget {
const UserProfileSubtitle({required this.subtitle, super.key});
final String subtitle;
@override
Widget build(BuildContext context) {
final theme = Theme.of(context);
return Padding(
padding: const EdgeInsets.fromLTRB(
AppSpacing.lg,
AppSpacing.sm,
AppSpacing.lg,
AppSpacing.md,
),
child: Text(
subtitle,
style: theme.textTheme.titleSmall,
),
);
}
}
@visibleForTesting
class UserProfileItem extends StatelessWidget {
const UserProfileItem({
required this.title,
this.leading,
this.trailing,
this.onTap,
super.key,
});
static const _leadingWidth = AppSpacing.xxxlg + AppSpacing.sm;
final String title;
final Widget? leading;
final Widget? trailing;
final VoidCallback? onTap;
@override
Widget build(BuildContext context) {
final hasLeading = leading != null;
return ListTile(
dense: true,
leading: SizedBox(
width: hasLeading ? _leadingWidth : 0,
child: leading,
),
trailing: trailing,
visualDensity: const VisualDensity(
vertical: VisualDensity.minimumDensity,
),
contentPadding: EdgeInsets.fromLTRB(
hasLeading ? 0 : AppSpacing.xlg,
AppSpacing.lg,
AppSpacing.lg,
AppSpacing.lg,
),
horizontalTitleGap: 0,
minLeadingWidth: hasLeading ? _leadingWidth : 0,
onTap: onTap,
title: Text(
title,
style: Theme.of(context).textTheme.titleMedium?.copyWith(
color: AppColors.highEmphasisSurface,
),
),
);
}
}
@visibleForTesting
class UserProfileLogoutButton extends StatelessWidget {
const UserProfileLogoutButton({super.key});
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.symmetric(
horizontal: AppSpacing.xxlg + AppSpacing.lg,
),
child: AppButton.smallDarkAqua(
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Assets.icons.logOutIcon.svg(),
const SizedBox(width: AppSpacing.sm),
Text(context.l10n.userProfileLogoutButtonText),
],
),
onPressed: () =>
context.read<AppBloc>().add(const AppLogoutRequested()),
),
);
}
}
class _UserProfileDivider extends StatelessWidget {
const _UserProfileDivider();
@override
Widget build(BuildContext context) {
return const Padding(
padding: EdgeInsets.symmetric(horizontal: AppSpacing.lg),
child: Divider(
color: AppColors.borderOutline,
indent: 0,
endIndent: 0,
),
);
}
}
| news_toolkit/flutter_news_example/lib/user_profile/view/user_profile_page.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/lib/user_profile/view/user_profile_page.dart",
"repo_id": "news_toolkit",
"token_count": 5099
} | 864 |
export 'analytics_event.dart';
export 'ntg_event.dart';
| news_toolkit/flutter_news_example/packages/analytics_repository/lib/src/models/models.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/analytics_repository/lib/src/models/models.dart",
"repo_id": "news_toolkit",
"token_count": 22
} | 865 |
import 'package:app_ui/app_ui.dart';
import 'package:flutter/material.dart';
class AppTextFieldPage extends StatelessWidget {
const AppTextFieldPage({super.key});
static Route<void> route() {
return MaterialPageRoute<void>(builder: (_) => const AppTextFieldPage());
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
'Text Field',
),
),
body: Padding(
padding: const EdgeInsets.all(AppSpacing.lg),
child: Column(
children: [
const AppEmailTextField(),
AppTextField(
hintText: 'Default text field',
onChanged: (_) {},
),
],
),
),
);
}
}
| news_toolkit/flutter_news_example/packages/app_ui/gallery/lib/widgets/app_text_field_page.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/app_ui/gallery/lib/widgets/app_text_field_page.dart",
"repo_id": "news_toolkit",
"token_count": 355
} | 866 |
import 'package:flutter/material.dart';
/// Modal which is styled for the Flutter News Example app.
Future<T?> showAppModal<T>({
required BuildContext context,
required WidgetBuilder builder,
RouteSettings? routeSettings,
BoxConstraints? constraints,
double? elevation,
Color? barrierColor,
bool isDismissible = true,
bool enableDrag = true,
AnimationController? transitionAnimationController,
}) {
return showModalBottomSheet(
context: context,
builder: builder,
routeSettings: routeSettings,
constraints: constraints,
isScrollControlled: true,
barrierColor: barrierColor,
isDismissible: isDismissible,
enableDrag: enableDrag,
transitionAnimationController: transitionAnimationController,
elevation: elevation,
);
}
| news_toolkit/flutter_news_example/packages/app_ui/lib/src/widgets/show_app_modal.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/app_ui/lib/src/widgets/show_app_modal.dart",
"repo_id": "news_toolkit",
"token_count": 235
} | 867 |
name: authentication_client
description: An Authentication Client Interface
publish_to: none
environment:
sdk: ">=3.0.0 <4.0.0"
dependencies:
equatable: ^2.0.3
dev_dependencies:
test: ^1.21.4
very_good_analysis: ^5.1.0
| news_toolkit/flutter_news_example/packages/authentication_client/authentication_client/pubspec.yaml/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/authentication_client/authentication_client/pubspec.yaml",
"repo_id": "news_toolkit",
"token_count": 91
} | 868 |
// ignore_for_file: prefer_const_constructors
import 'package:mocktail/mocktail.dart';
import 'package:test/test.dart';
import 'package:token_storage/token_storage.dart';
// TokenStorage is exported and can be implemented.
class FakeTokenStorage extends Fake implements TokenStorage {}
void main() {
group('TokenStorage', () {
test('TokenStorage can be implemented', () {
expect(FakeTokenStorage.new, returnsNormally);
});
});
group('InMemoryTokenStorage', () {
test(
'readToken returns null '
'when no token is saved', () async {
final storage = InMemoryTokenStorage();
expect(await storage.readToken(), isNull);
});
test(
'readToken returns token '
'when token is saved with saveToken', () async {
const token = 'token';
final storage = InMemoryTokenStorage();
await storage.saveToken(token);
expect(await storage.readToken(), equals(token));
});
test(
'readToken returns updated token '
'when token is overridden with saveToken', () async {
const token = 'token';
const updatedToken = 'updatedToken';
final storage = InMemoryTokenStorage();
await storage.saveToken(token);
expect(await storage.readToken(), equals(token));
await storage.saveToken(updatedToken);
expect(await storage.readToken(), equals(updatedToken));
});
test('clearToken clears token', () async {
const token = 'token';
final storage = InMemoryTokenStorage();
await storage.saveToken(token);
await storage.clearToken();
expect(await storage.readToken(), isNull);
});
});
}
| news_toolkit/flutter_news_example/packages/authentication_client/token_storage/test/src/token_storage_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/authentication_client/token_storage/test/src/token_storage_test.dart",
"repo_id": "news_toolkit",
"token_count": 575
} | 869 |
include: package:very_good_analysis/analysis_options.5.1.0.yaml
| news_toolkit/flutter_news_example/packages/form_inputs/analysis_options.yaml/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/form_inputs/analysis_options.yaml",
"repo_id": "news_toolkit",
"token_count": 23
} | 870 |
<svg width="16" height="16" viewBox="0 0 16 16" fill="none" xmlns="http://www.w3.org/2000/svg">
<path d="M16 7H3.83L9.42 1.41L8 0L0 8L8 16L9.41 14.59L3.83 9H16V7Z" fill="white"/>
</svg>
| news_toolkit/flutter_news_example/packages/news_blocks_ui/assets/icons/arrow_left_disable.svg/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/news_blocks_ui/assets/icons/arrow_left_disable.svg",
"repo_id": "news_toolkit",
"token_count": 96
} | 871 |
import 'package:app_ui/app_ui.dart';
import 'package:flutter/material.dart';
import 'package:news_blocks_ui/src/newsletter/index.dart';
/// {@template newsletter_sign_up}
/// A reusable newsletter news block widget.
/// {@endtemplate}
class NewsletterSignUp extends StatelessWidget {
/// {@macro newsletter_sign_up}
const NewsletterSignUp({
required this.headerText,
required this.bodyText,
required this.email,
required this.buttonText,
required this.onPressed,
super.key,
});
/// The header displayed message.
final String headerText;
/// The body displayed message.
final String bodyText;
/// The header displayed message.
final Widget email;
/// The text displayed in button.
final String buttonText;
/// The callback which is invoked when the button is pressed.
final VoidCallback? onPressed;
@override
Widget build(BuildContext context) {
final theme = Theme.of(context);
return NewsletterContainer(
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
Text(
headerText,
textAlign: TextAlign.center,
style: theme.textTheme.headlineMedium
?.copyWith(color: AppColors.highEmphasisPrimary),
),
const SizedBox(height: AppSpacing.lg),
Text(
bodyText,
textAlign: TextAlign.center,
style: theme.textTheme.bodyLarge
?.copyWith(color: AppColors.mediumEmphasisPrimary),
),
const SizedBox(height: AppSpacing.lg),
email,
AppButton.secondary(
onPressed: onPressed,
textStyle: theme.textTheme.labelLarge?.copyWith(
color: AppColors.white,
),
child: Text(
buttonText,
),
),
],
),
);
}
}
| news_toolkit/flutter_news_example/packages/news_blocks_ui/lib/src/newsletter/newsletter_sign_up.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/news_blocks_ui/lib/src/newsletter/newsletter_sign_up.dart",
"repo_id": "news_toolkit",
"token_count": 802
} | 872 |
import 'package:app_ui/app_ui.dart';
import 'package:flutter/material.dart';
import 'package:news_blocks/news_blocks.dart';
/// {@template text_caption}
/// A reusable text caption news block widget.
/// {@endtemplate}
class TextCaption extends StatelessWidget {
/// {@macro text_caption}
const TextCaption({
required this.block,
this.colorValues = _defaultColorValues,
super.key,
});
/// The associated [TextCaption] instance.
final TextCaptionBlock block;
/// The color values of this text caption.
///
/// Defaults to [_defaultColorValues].
final Map<TextCaptionColor, Color> colorValues;
/// The default color values of this text caption.
static const _defaultColorValues = <TextCaptionColor, Color>{
TextCaptionColor.normal: AppColors.highEmphasisSurface,
TextCaptionColor.light: AppColors.mediumEmphasisSurface,
};
@override
Widget build(BuildContext context) {
final color = colorValues.containsKey(block.color)
? colorValues[block.color]
: AppColors.highEmphasisSurface;
return Padding(
padding: const EdgeInsets.symmetric(horizontal: AppSpacing.lg),
child: Text(
block.text,
style: Theme.of(context).textTheme.bodySmall?.apply(color: color),
),
);
}
}
| news_toolkit/flutter_news_example/packages/news_blocks_ui/lib/src/text_caption.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/news_blocks_ui/lib/src/text_caption.dart",
"repo_id": "news_toolkit",
"token_count": 445
} | 873 |
import 'package:app_ui/app_ui.dart';
import 'package:flutter/material.dart';
/// {@template post_content_premium_category}
/// A widget displaying a premium label on a post.
/// {@endtemplate}
class PostContentPremiumCategory extends StatelessWidget {
/// {@macro post_content_premium_category}
const PostContentPremiumCategory({
required this.premiumText,
required this.isVideoContent,
super.key,
});
/// Text displayed when post is premium content.
final String premiumText;
/// Whether content is a part of a video article.
final bool isVideoContent;
@override
Widget build(BuildContext context) {
const backgroundColor = AppColors.redWine;
const textColor = AppColors.white;
const horizontalSpacing = AppSpacing.xs;
return Column(
children: [
Align(
alignment: Alignment.centerLeft,
child: DecoratedBox(
decoration: const BoxDecoration(color: backgroundColor),
child: Padding(
padding: const EdgeInsets.fromLTRB(
horizontalSpacing,
0,
horizontalSpacing,
AppSpacing.xxs,
),
child: Text(
premiumText.toUpperCase(),
style: Theme.of(context)
.textTheme
.labelSmall
?.copyWith(color: textColor),
),
),
),
),
const SizedBox(height: AppSpacing.sm),
],
);
}
}
| news_toolkit/flutter_news_example/packages/news_blocks_ui/lib/src/widgets/post_content_premium_category.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/news_blocks_ui/lib/src/widgets/post_content_premium_category.dart",
"repo_id": "news_toolkit",
"token_count": 684
} | 874 |
// ignore_for_file: unnecessary_const, prefer_const_constructors
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:news_blocks/news_blocks.dart';
import 'package:news_blocks_ui/news_blocks_ui.dart';
import '../helpers/helpers.dart';
void main() {
group('DividerHorizontal', () {
setUpAll(setUpTolerantComparator);
testWidgets('renders correctly', (tester) async {
final widget = Center(
child: DividerHorizontal(
block: DividerHorizontalBlock(),
),
);
await tester.pumpApp(widget);
await expectLater(
find.byType(DividerHorizontal),
matchesGoldenFile('divider_horizontal.png'),
);
});
});
}
| news_toolkit/flutter_news_example/packages/news_blocks_ui/test/src/divider_horizontal_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/news_blocks_ui/test/src/divider_horizontal_test.dart",
"repo_id": "news_toolkit",
"token_count": 293
} | 875 |
// ignore_for_file: prefer_const_constructors
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:mocktail_image_network/mocktail_image_network.dart';
import 'package:news_blocks/news_blocks.dart';
import 'package:news_blocks_ui/news_blocks_ui.dart';
import '../../helpers/helpers.dart';
void main() {
setUpAll(() {
setUpTolerantComparator();
setUpMockPathProvider();
});
group('PostMedium', () {
const id = '82c49bf1-946d-4920-a801-302291f367b5';
const category = PostCategory.sports;
const author = 'Tom Dierberger';
final publishedAt = DateTime(2022, 3, 10);
const imageUrl =
'https://www.nbcsports.com/sites/rsnunited/files/styles/metatags_opengraph/public/article/hero/pat-bev-ja-morant-USA.jpg';
const title = 'No Man’s Sky’s new Outlaws update '
'lets players go full space pirate';
const description =
'No Man’s Sky’s newest update, Outlaws, is now live, and it lets '
'players find and smuggle black market goods and evade the '
'authorities in outlaw systems.';
testWidgets('renders correctly overlaid layout', (tester) async {
final postMediumBlock = PostMediumBlock(
id: id,
category: category,
author: author,
publishedAt: publishedAt,
imageUrl: imageUrl,
title: title,
description: description,
isContentOverlaid: true,
);
await mockNetworkImages(
() async => tester.pumpContentThemedApp(
Column(children: [PostMedium(block: postMediumBlock)]),
),
);
expect(
find.byType(PostMediumOverlaidLayout),
matchesGoldenFile('post_medium_overlaid_layout.png'),
);
});
testWidgets('renders correctly description layout', (tester) async {
final postMediumBlock = PostMediumBlock(
id: id,
category: category,
author: author,
publishedAt: publishedAt,
imageUrl: imageUrl,
title: title,
description: description,
);
await mockNetworkImages(
() async => tester.pumpContentThemedApp(
Column(children: [PostMedium(block: postMediumBlock)]),
),
);
expect(
find.byType(PostMediumDescriptionLayout),
matchesGoldenFile('post_medium_description_layout.png'),
);
});
testWidgets('onPressed is called with action when tapped', (tester) async {
final action = NavigateToArticleAction(articleId: id);
final actions = <BlockAction>[];
final postMediumBlock = PostMediumBlock(
id: id,
category: category,
author: author,
publishedAt: publishedAt,
imageUrl: imageUrl,
title: title,
description: description,
action: action,
);
await mockNetworkImages(
() async => tester.pumpContentThemedApp(
PostMedium(
block: postMediumBlock,
onPressed: actions.add,
),
),
);
final widget = find.byType(PostMediumDescriptionLayout);
expect(widget, findsOneWidget);
await tester.tap(widget);
expect(actions, [action]);
});
});
}
| news_toolkit/flutter_news_example/packages/news_blocks_ui/test/src/post_medium/post_medium_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/news_blocks_ui/test/src/post_medium/post_medium_test.dart",
"repo_id": "news_toolkit",
"token_count": 1334
} | 876 |
// ignore_for_file: unnecessary_const, prefer_const_constructors
import 'package:flutter_test/flutter_test.dart';
import 'package:mocktail_image_network/mocktail_image_network.dart';
import 'package:news_blocks/news_blocks.dart';
import 'package:news_blocks_ui/news_blocks_ui.dart';
import '../helpers/helpers.dart';
void main() {
group('TrendingStory', () {
setUpAll(() {
setUpTolerantComparator();
setUpMockPathProvider();
});
testWidgets('renders correctly', (tester) async {
await mockNetworkImages(() async {
final widget = TrendingStory(
title: 'TRENDING',
block: TrendingStoryBlock(
content: PostSmallBlock(
id: 'id',
category: PostCategory.technology,
author: 'author',
publishedAt: DateTime(2022, 3, 11),
imageUrl: 'imageUrl',
title: 'title',
),
),
);
await tester.pumpApp(widget);
await expectLater(
find.byType(TrendingStory),
matchesGoldenFile('trending_story.png'),
);
});
});
});
}
| news_toolkit/flutter_news_example/packages/news_blocks_ui/test/src/trending_story_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/news_blocks_ui/test/src/trending_story_test.dart",
"repo_id": "news_toolkit",
"token_count": 525
} | 877 |
export 'src/firebase_notifications_client.dart';
| news_toolkit/flutter_news_example/packages/notifications_client/firebase_notifications_client/lib/firebase_notifications_client.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/notifications_client/firebase_notifications_client/lib/firebase_notifications_client.dart",
"repo_id": "news_toolkit",
"token_count": 16
} | 878 |
name: one_signal_notifications_client
description: OneSignal notifications client.
version: 1.0.0+1
publish_to: none
environment:
sdk: ">=3.0.0 <4.0.0"
dependencies:
flutter:
sdk: flutter
notifications_client:
path: ../notifications_client
onesignal_flutter: ^3.4.0
dev_dependencies:
flutter_test:
sdk: flutter
mocktail: ^1.0.2
very_good_analysis: ^5.1.0
| news_toolkit/flutter_news_example/packages/notifications_client/one_signal_notifications_client/pubspec.yaml/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/notifications_client/one_signal_notifications_client/pubspec.yaml",
"repo_id": "news_toolkit",
"token_count": 162
} | 879 |
name: package_info_client
description: A client that provides information about the app's package metadata
version: 1.0.0+1
publish_to: none
environment:
sdk: ">=3.0.0 <4.0.0"
dependencies:
equatable: ^2.0.3
dev_dependencies:
test: ^1.21.4
very_good_analysis: ^5.1.0
| news_toolkit/flutter_news_example/packages/package_info_client/pubspec.yaml/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/package_info_client/pubspec.yaml",
"repo_id": "news_toolkit",
"token_count": 110
} | 880 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter_test/flutter_test.dart';
import 'package:in_app_purchase/in_app_purchase.dart';
import 'package:purchase_client/purchase_client.dart';
import 'package:purchase_client/src/products.dart';
extension _PurchaseDetailsEquals on PurchaseDetails {
/// Returns a copy of the current PurchaseDetails with the given parameters.
bool equals(PurchaseDetails purchaseDetails) =>
purchaseDetails.purchaseID == purchaseID &&
purchaseDetails.productID == productID &&
purchaseDetails.verificationData == verificationData &&
purchaseDetails.transactionDate == transactionDate &&
purchaseDetails.status == status &&
purchaseDetails.pendingCompletePurchase == pendingCompletePurchase &&
purchaseDetails.error == error;
}
void main() {
group('PurchaseDetails', () {
group('copyWith', () {
final purchaseDetails = PurchaseDetails(
productID: 'id',
status: PurchaseStatus.pending,
transactionDate: 'date',
verificationData: PurchaseVerificationData(
localVerificationData: 'local',
serverVerificationData: 'server',
source: 'source',
),
);
test(
'returns the same object '
'when no parameters are passed', () {
expect(purchaseDetails.copyWith().equals(purchaseDetails), isTrue);
});
test(
'sets purchaseID '
'when purchaseID is passed', () {
expect(
purchaseDetails.copyWith(purchaseID: 'newId').purchaseID,
'newId',
);
});
test(
'sets productID '
'when productID is passed', () {
expect(purchaseDetails.copyWith(productID: 'newId').productID, 'newId');
});
test(
'sets status '
'when status is passed', () {
expect(
purchaseDetails.copyWith(status: PurchaseStatus.purchased).status,
PurchaseStatus.purchased,
);
});
test(
'sets transactionDate '
'when transactionDate is passed', () {
expect(
purchaseDetails.copyWith(transactionDate: 'newDate').transactionDate,
'newDate',
);
});
test(
'sets verificationData '
'when verificationData is passed', () {
final verificationData = PurchaseVerificationData(
localVerificationData: 'newLocal',
serverVerificationData: 'newServer',
source: 'newSource',
);
expect(
purchaseDetails
.copyWith(verificationData: verificationData)
.verificationData,
verificationData,
);
});
test(
'sets pendingCompletePurchase '
'when pendingCompletePurchase is passed', () {
expect(
purchaseDetails
.copyWith(pendingCompletePurchase: true)
.pendingCompletePurchase,
isTrue,
);
});
});
});
group('PurchaseClient', () {
final productDetails = availableProducts.values.first;
final purchaseDetails = PurchaseDetails(
productID: 'productID',
verificationData: PurchaseVerificationData(
localVerificationData: 'localVerificationData',
serverVerificationData: 'serverVerificationData',
source: 'local',
),
transactionDate: 'transactionDate',
status: PurchaseStatus.purchased,
);
late PurchaseClient purchaseClient;
setUp(() {
purchaseClient = PurchaseClient();
});
test('isAvailable returns true', () {
expect(purchaseClient.isAvailable(), completion(isTrue));
});
group('queryProductDetails', () {
test(
'returns empty productDetails '
'with an empty notFoundIDs '
'when no identifiers are provided', () async {
final response = await purchaseClient.queryProductDetails(<String>{});
expect(response.notFoundIDs, isEmpty);
expect(response.productDetails, isEmpty);
});
test(
'returns found productDetails '
'with an empty notFoundIDs', () async {
final response = await purchaseClient
.queryProductDetails(<String>{productDetails.id});
expect(response.notFoundIDs, isEmpty);
expect(response.productDetails.length, equals(1));
expect(response.productDetails.first.id, equals(productDetails.id));
});
test(
'returns an empty productDetails '
'with provided id in notFoundIDs', () async {
final response =
await purchaseClient.queryProductDetails(<String>{'unknownId'});
expect(response.notFoundIDs, equals(['unknownId']));
expect(response.productDetails, equals(<ProductDetails>[]));
});
});
test(
'buyNonConsumable returns true '
'and adds purchase '
'with purchase status PurchaseStatus.pending '
'to purchaseStream', () async {
final result = await purchaseClient.buyNonConsumable(
purchaseParam: PurchaseParam(
productDetails: productDetails,
applicationUserName: 'testUserName',
),
);
purchaseClient.purchaseStream.listen((purchases) {
expect(purchases, equals([purchaseDetails]));
expect(purchases.first.status, equals(PurchaseStatus.pending));
});
expect(result, true);
});
test(
'completePurchase adds purchaseDetails to purchaseStream '
'with pendingCompletePurchase set to false', () async {
await Future.wait([
expectLater(
purchaseClient.purchaseStream,
emitsThrough(
(List<PurchaseDetails> purchases) => purchases.first.equals(
purchaseDetails.copyWith(
pendingCompletePurchase: false,
),
),
),
),
purchaseClient.completePurchase(purchaseDetails),
]);
});
test('restorePurchases completes', () async {
await expectLater(purchaseClient.restorePurchases(), completes);
});
test('buyConsumable throws an UnimplementedError', () async {
expect(
() => purchaseClient.buyConsumable(
purchaseParam: PurchaseParam(
productDetails: productDetails,
applicationUserName: 'testUserName',
),
),
throwsA(isA<UnimplementedError>()),
);
});
test('getPlatformAddition throws an UnimplementedError', () async {
expect(
() => purchaseClient.getPlatformAddition(),
throwsA(isA<UnimplementedError>()),
);
});
});
}
| news_toolkit/flutter_news_example/packages/purchase_client/test/src/purchase_client_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/purchase_client/test/src/purchase_client_test.dart",
"repo_id": "news_toolkit",
"token_count": 2734
} | 881 |
include: package:very_good_analysis/analysis_options.5.1.0.yaml
| news_toolkit/flutter_news_example/packages/storage/secure_storage/analysis_options.yaml/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/storage/secure_storage/analysis_options.yaml",
"repo_id": "news_toolkit",
"token_count": 23
} | 882 |
part of 'user_repository.dart';
/// Storage keys for the [UserStorage].
abstract class UserStorageKeys {
/// Number of times that a user opened the application.
static const appOpenedCount = '__app_opened_count_key__';
}
/// {@template user_storage}
/// Storage for the [UserRepository].
/// {@endtemplate}
class UserStorage {
/// {@macro user_storage}
const UserStorage({
required Storage storage,
}) : _storage = storage;
final Storage _storage;
/// Sets the number of times the app was opened.
Future<void> setAppOpenedCount({required int count}) => _storage.write(
key: UserStorageKeys.appOpenedCount,
value: count.toString(),
);
/// Fetches the number of times the app was opened value from Storage.
Future<int> fetchAppOpenedCount() async {
final count = await _storage.read(key: UserStorageKeys.appOpenedCount);
return int.parse(count ?? '0');
}
}
| news_toolkit/flutter_news_example/packages/user_repository/lib/src/user_storage.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/packages/user_repository/lib/src/user_storage.dart",
"repo_id": "news_toolkit",
"token_count": 290
} | 883 |
// ignore_for_file: prefer_const_constructors, must_be_immutable
import 'package:flutter_news_example/app/app.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:mocktail/mocktail.dart';
import 'package:user_repository/user_repository.dart';
class MockUser extends Mock implements User {}
void main() {
group('AppEvent', () {
group('AppUserChanged', () {
final user = MockUser();
test('supports value comparisons', () {
expect(
AppUserChanged(user),
AppUserChanged(user),
);
});
});
group('AppOnboardingCompleted', () {
test('supports value comparisons', () {
expect(
AppOnboardingCompleted(),
AppOnboardingCompleted(),
);
});
});
group('AppLogoutRequested', () {
test('supports value comparisons', () {
expect(
AppLogoutRequested(),
AppLogoutRequested(),
);
});
});
group('AppOpened', () {
test('supports value comparisons', () {
expect(
AppOpened(),
AppOpened(),
);
});
});
});
}
| news_toolkit/flutter_news_example/test/app/bloc/app_event_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/test/app/bloc/app_event_test.dart",
"repo_id": "news_toolkit",
"token_count": 493
} | 884 |
// ignore_for_file: prefer_const_constructors
// ignore_for_file: prefer_const_literals_to_create_immutables
import 'package:bloc_test/bloc_test.dart';
import 'package:flutter_news_example/categories/categories.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:mocktail/mocktail.dart';
import 'package:news_repository/news_repository.dart';
import '../../helpers/helpers.dart';
class MockNewsRepository extends Mock implements NewsRepository {}
void main() {
initMockHydratedStorage();
group('CategoriesBloc', () {
late NewsRepository newsRepository;
late CategoriesBloc categoriesBloc;
final categoriesResponse = CategoriesResponse(
categories: [Category.top, Category.health],
);
setUp(() async {
newsRepository = MockNewsRepository();
categoriesBloc = CategoriesBloc(newsRepository: newsRepository);
});
test('can be (de)serialized', () {
final categoriesState = CategoriesState(
status: CategoriesStatus.populated,
categories: categoriesResponse.categories,
selectedCategory: categoriesResponse.categories.first,
);
final serialized = categoriesBloc.toJson(categoriesState);
final deserialized = categoriesBloc.fromJson(serialized!);
expect(deserialized, categoriesState);
});
group('CategoriesRequested', () {
blocTest<CategoriesBloc, CategoriesState>(
'emits [loading, populated] '
'when getCategories succeeds',
setUp: () => when(newsRepository.getCategories)
.thenAnswer((_) async => categoriesResponse),
build: () => categoriesBloc,
act: (bloc) => bloc.add(CategoriesRequested()),
expect: () => <CategoriesState>[
CategoriesState(status: CategoriesStatus.loading),
CategoriesState(
status: CategoriesStatus.populated,
categories: categoriesResponse.categories,
selectedCategory: categoriesResponse.categories.first,
),
],
);
blocTest<CategoriesBloc, CategoriesState>(
'emits [loading, failure] '
'when getCategories fails',
setUp: () => when(newsRepository.getCategories).thenThrow(Exception()),
build: () => categoriesBloc,
act: (bloc) => bloc.add(CategoriesRequested()),
expect: () => <CategoriesState>[
CategoriesState(status: CategoriesStatus.loading),
CategoriesState(status: CategoriesStatus.failure),
],
);
});
group('CategorySelected', () {
blocTest<CategoriesBloc, CategoriesState>(
'emits selectedCategory',
build: () => categoriesBloc,
act: (bloc) => bloc.add(CategorySelected(category: Category.top)),
expect: () => <CategoriesState>[
CategoriesState.initial().copyWith(
selectedCategory: Category.top,
),
],
);
});
});
}
| news_toolkit/flutter_news_example/test/categories/bloc/categories_bloc_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/test/categories/bloc/categories_bloc_test.dart",
"repo_id": "news_toolkit",
"token_count": 1112
} | 885 |
import 'package:bloc_test/bloc_test.dart';
import 'package:flutter_news_example/home/home.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
group('HomeCubit', () {
group('constructor', () {
test('has correct initial state', () async {
expect(
HomeCubit().state,
equals(HomeState.topStories),
);
});
});
group('setTab', () {
blocTest<HomeCubit, HomeState>(
'sets tab on top stories',
build: HomeCubit.new,
act: (cubit) => cubit.setTab(0),
expect: () => [
HomeState.topStories,
],
);
blocTest<HomeCubit, HomeState>(
'sets tab on search',
build: HomeCubit.new,
act: (cubit) => cubit.setTab(1),
expect: () => [
HomeState.search,
],
);
blocTest<HomeCubit, HomeState>(
'sets tab on subscribe',
build: HomeCubit.new,
act: (cubit) => cubit.setTab(2),
expect: () => [
HomeState.subscribe,
],
);
});
});
}
| news_toolkit/flutter_news_example/test/home/cubit/home_cubit_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/test/home/cubit/home_cubit_test.dart",
"repo_id": "news_toolkit",
"token_count": 531
} | 886 |
import 'package:analytics_repository/analytics_repository.dart';
import 'package:bloc/bloc.dart';
import 'package:equatable/equatable.dart';
import 'package:flutter_bloc/flutter_bloc.dart';
import 'package:flutter_news_example/main/bootstrap/app_bloc_observer.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:mocktail/mocktail.dart';
class MockAnalyticsRepository extends Mock implements AnalyticsRepository {}
class FakeBloc extends Fake implements Bloc<dynamic, dynamic> {}
class TestAnalyticsEvent extends Equatable with AnalyticsEventMixin {
@override
AnalyticsEvent get event => const AnalyticsEvent('TestAnalyticsEvent');
}
class FakeAnalyticsEvent extends Fake implements AnalyticsEvent {}
void main() {
group('AppBlocObserver', () {
late Bloc<dynamic, dynamic> bloc;
late AnalyticsRepository analyticsRepository;
late AppBlocObserver observer;
setUp(() {
bloc = FakeBloc();
analyticsRepository = MockAnalyticsRepository();
when(() => analyticsRepository.track(any())).thenAnswer((_) async {});
observer = AppBlocObserver(analyticsRepository: analyticsRepository);
});
setUpAll(() {
registerFallbackValue(FakeAnalyticsEvent());
});
group('onTransition', () {
test('returns normally', () {
final transition = Transition(
currentState: TestAnalyticsEvent(),
event: TestAnalyticsEvent(),
nextState: TestAnalyticsEvent(),
);
expect(() => observer.onTransition(bloc, transition), returnsNormally);
});
});
group('onChange', () {
test('tracks analytics event', () {
final change = Change(
currentState: TestAnalyticsEvent(),
nextState: TestAnalyticsEvent(),
);
expect(() => observer.onChange(bloc, change), returnsNormally);
verify(
() => analyticsRepository.track(change.nextState.event),
).called(1);
});
});
group('onEvent', () {
test('tracks analytics event', () {
final event = TestAnalyticsEvent();
expect(() => observer.onEvent(bloc, event), returnsNormally);
verify(() => analyticsRepository.track(event.event)).called(1);
});
});
group('onError', () {
test('returns normally', () {
final error = Exception();
const stackTrace = StackTrace.empty;
expect(
() => observer.onError(bloc, error, stackTrace),
returnsNormally,
);
});
});
});
}
| news_toolkit/flutter_news_example/test/main/bootstrap/app_bloc_observer_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/test/main/bootstrap/app_bloc_observer_test.dart",
"repo_id": "news_toolkit",
"token_count": 940
} | 887 |
// ignore_for_file: prefer_const_constructors
import 'package:ads_consent_client/ads_consent_client.dart';
import 'package:bloc_test/bloc_test.dart';
import 'package:flutter_news_example/onboarding/onboarding.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:mocktail/mocktail.dart';
import 'package:notifications_repository/notifications_repository.dart';
class MockNotificationsRepository extends Mock
implements NotificationsRepository {}
class MockAdsConsentClient extends Mock implements AdsConsentClient {}
void main() {
group('OnboardingBloc', () {
late NotificationsRepository notificationsRepository;
late AdsConsentClient adsConsentClient;
setUp(() {
notificationsRepository = MockNotificationsRepository();
adsConsentClient = MockAdsConsentClient();
});
group('EnableAdTrackingRequested', () {
blocTest<OnboardingBloc, OnboardingState>(
'emits '
'[EnablingAdTracking, EnablingAdTrackingSucceeded] '
'when AdsConsentClient.requestConsent returns true',
setUp: () =>
when(adsConsentClient.requestConsent).thenAnswer((_) async => true),
build: () => OnboardingBloc(
notificationsRepository: notificationsRepository,
adsConsentClient: adsConsentClient,
),
act: (bloc) => bloc.add(EnableAdTrackingRequested()),
expect: () => <OnboardingState>[
EnablingAdTracking(),
EnablingAdTrackingSucceeded(),
],
verify: (bloc) => verify(adsConsentClient.requestConsent).called(1),
);
blocTest<OnboardingBloc, OnboardingState>(
'emits '
'[EnablingAdTracking, EnablingAdTrackingFailed] '
'when AdsConsentClient.requestConsent returns false',
setUp: () => when(adsConsentClient.requestConsent)
.thenAnswer((_) async => false),
build: () => OnboardingBloc(
notificationsRepository: notificationsRepository,
adsConsentClient: adsConsentClient,
),
act: (bloc) => bloc.add(EnableAdTrackingRequested()),
expect: () => <OnboardingState>[
EnablingAdTracking(),
EnablingAdTrackingFailed(),
],
verify: (bloc) => verify(adsConsentClient.requestConsent).called(1),
);
blocTest<OnboardingBloc, OnboardingState>(
'emits '
'[EnablingNotifications, EnablingNotificationsFailed] '
'when AdsConsentClient.requestConsent fails',
setUp: () =>
when(adsConsentClient.requestConsent).thenThrow(Exception()),
build: () => OnboardingBloc(
notificationsRepository: notificationsRepository,
adsConsentClient: adsConsentClient,
),
act: (bloc) => bloc.add(EnableAdTrackingRequested()),
expect: () => <OnboardingState>[
EnablingAdTracking(),
EnablingAdTrackingFailed(),
],
);
});
group('EnableNotificationsRequested', () {
setUp(() {
when(
() => notificationsRepository.toggleNotifications(
enable: any(named: 'enable'),
),
).thenAnswer((_) async {});
});
blocTest<OnboardingBloc, OnboardingState>(
'emits '
'[EnablingNotifications, EnablingNotificationsSucceeded] '
'when NotificationsRepository.toggleNotifications succeeds',
build: () => OnboardingBloc(
notificationsRepository: notificationsRepository,
adsConsentClient: adsConsentClient,
),
act: (bloc) => bloc.add(EnableNotificationsRequested()),
expect: () => <OnboardingState>[
EnablingNotifications(),
EnablingNotificationsSucceeded(),
],
verify: (bloc) => verify(
() => notificationsRepository.toggleNotifications(enable: true),
).called(1),
);
blocTest<OnboardingBloc, OnboardingState>(
'emits '
'[EnablingNotifications, EnablingNotificationsFailed] '
'when NotificationsRepository.toggleNotifications fails',
setUp: () => when(
() => notificationsRepository.toggleNotifications(
enable: any(named: 'enable'),
),
).thenThrow(Exception()),
build: () => OnboardingBloc(
notificationsRepository: notificationsRepository,
adsConsentClient: adsConsentClient,
),
act: (bloc) => bloc.add(EnableNotificationsRequested()),
expect: () => <OnboardingState>[
EnablingNotifications(),
EnablingNotificationsFailed(),
],
);
});
});
}
| news_toolkit/flutter_news_example/test/onboarding/bloc/onboarding_bloc_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/test/onboarding/bloc/onboarding_bloc_test.dart",
"repo_id": "news_toolkit",
"token_count": 1889
} | 888 |
import 'package:flutter_news_example/subscriptions/subscriptions.dart';
// ignore: lines_longer_than_80_chars
// ignore_for_file: prefer_const_literals_to_create_immutables, prefer_const_constructors
import 'package:flutter_test/flutter_test.dart';
import 'package:in_app_purchase_repository/in_app_purchase_repository.dart';
void main() {
group('SubscriptionsState', () {
test('initial has correct status', () {
final initialState = SubscriptionsState.initial();
expect(
initialState,
equals(
SubscriptionsState(
subscriptions: [],
purchaseStatus: PurchaseStatus.none,
),
),
);
});
test('supports value comparisons', () {
expect(
SubscriptionsState.initial(),
equals(
SubscriptionsState.initial(),
),
);
});
group('copyWith', () {
test(
'returns same object '
'when no parameters changed', () {
expect(
SubscriptionsState.initial().copyWith(),
equals(SubscriptionsState.initial()),
);
});
test(
'returns object with updated purchaseStatus '
'when status changed', () {
expect(
SubscriptionsState.initial().copyWith(
purchaseStatus: PurchaseStatus.completed,
),
equals(
SubscriptionsState(
subscriptions: [],
purchaseStatus: PurchaseStatus.completed,
),
),
);
});
test(
'returns object with updated subscriptions '
'when subscriptions changed', () {
expect(
SubscriptionsState.initial().copyWith(
subscriptions: [
Subscription(
benefits: [],
cost: SubscriptionCost(
annual: 0,
monthly: 0,
),
id: '1',
name: SubscriptionPlan.none,
),
],
),
equals(
SubscriptionsState(
subscriptions: [
Subscription(
benefits: [],
cost: SubscriptionCost(
annual: 0,
monthly: 0,
),
id: '1',
name: SubscriptionPlan.none,
),
],
purchaseStatus: PurchaseStatus.none,
),
),
);
});
});
});
}
| news_toolkit/flutter_news_example/test/subscriptions/dialog/bloc/subscriptions_state_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/test/subscriptions/dialog/bloc/subscriptions_state_test.dart",
"repo_id": "news_toolkit",
"token_count": 1317
} | 889 |
// ignore_for_file: prefer_const_constructors
import 'dart:async';
import 'package:flutter/material.dart';
import 'package:flutter_news_example/user_profile/user_profile.dart';
import 'package:flutter_test/flutter_test.dart';
import '../../helpers/helpers.dart';
void main() {
group('UserProfileSubscribeBox', () {
testWidgets('calls onSubscribePressed when AppButton tapped',
(tester) async {
final completer = Completer<void>();
await tester.pumpApp(
UserProfileSubscribeBox(onSubscribePressed: completer.complete),
);
await tester.tap(find.byKey(Key('userProfileSubscribeBox_appButton')));
expect(completer.isCompleted, isTrue);
});
});
}
| news_toolkit/flutter_news_example/test/user_profile/widgets/user_profile_subscribe_box_test.dart/0 | {
"file_path": "news_toolkit/flutter_news_example/test/user_profile/widgets/user_profile_subscribe_box_test.dart",
"repo_id": "news_toolkit",
"token_count": 263
} | 890 |
#!/bin/bash
# Copyright 2013 The Flutter Authors. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
set -e
# Ensure that the create/boot pipeline fails if `create` fails
set -o pipefail
# The name here must match remove_simulator.sh
readonly DEVICE_NAME=Flutter-iPhone
readonly DEVICE=com.apple.CoreSimulator.SimDeviceType.iPhone-14
readonly OS=com.apple.CoreSimulator.SimRuntime.iOS-17-0
# Delete any existing devices named Flutter-iPhone. Having more than one may
# cause issues when builds target the device.
echo -e "Deleting any existing devices names $DEVICE_NAME..."
RESULT=0
while [[ $RESULT == 0 ]]; do
xcrun simctl delete "$DEVICE_NAME" || RESULT=1
if [ $RESULT == 0 ]; then
echo -e "Deleted $DEVICE_NAME"
fi
done
echo -e ""
echo -e "\nCreating $DEVICE_NAME $DEVICE $OS ...\n"
xcrun simctl create "$DEVICE_NAME" "$DEVICE" "$OS" | xargs xcrun simctl boot
xcrun simctl list
| packages/.ci/scripts/create_simulator.sh/0 | {
"file_path": "packages/.ci/scripts/create_simulator.sh",
"repo_id": "packages",
"token_count": 324
} | 891 |
tasks:
- name: prepare tool
script: .ci/scripts/prepare_tool.sh
infra_step: true # Note infra steps failing prevents "always" from running.
- name: create all_packages app
script: .ci/scripts/create_all_packages_app.sh
infra_step: true # Note infra steps failing prevents "always" from running.
- name: build all_packages for Android debug
script: .ci/scripts/build_all_packages_app.sh
args: ["apk", "debug"]
- name: build all_packages for Android release
script: .ci/scripts/build_all_packages_app.sh
args: ["apk", "release"]
- name: create all_packages app - legacy version
script: .ci/scripts/create_all_packages_app_legacy.sh
# Output dir; must match the final argument to build_all_packages_app_legacy
# below.
args: ["legacy"]
# Only build legacy in one mode, to minimize extra CI time. Debug is chosen
# somewhat arbitrarily as likely being slightly faster.
- name: build all_packages for Android - legacy version
script: .ci/scripts/build_all_packages_app_legacy.sh
# The final argument here must match the output directory passed to
# create_all_packages_app_legacy above.
args: ["apk", "debug", "legacy"]
| packages/.ci/targets/android_build_all_packages.yaml/0 | {
"file_path": "packages/.ci/targets/android_build_all_packages.yaml",
"repo_id": "packages",
"token_count": 388
} | 892 |
tasks:
- name: prepare tool
script: .ci/scripts/prepare_tool.sh
infra_step: true # Note infra steps failing prevents "always" from running.
- name: tool unit tests
script: .ci/scripts/plugin_tools_tests.sh
| packages/.ci/targets/repo_tools_tests.yaml/0 | {
"file_path": "packages/.ci/targets/repo_tools_tests.yaml",
"repo_id": "packages",
"token_count": 77
} | 893 |
buildFlags:
_pluginToolsConfigGlobalKey:
- "--no-tree-shake-icons"
- "--dart-define=buildmode=testing"
| packages/packages/animations/example/android/.pluginToolsConfig.yaml/0 | {
"file_path": "packages/packages/animations/example/android/.pluginToolsConfig.yaml",
"repo_id": "packages",
"token_count": 45
} | 894 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:ui' as ui;
import 'package:animations/src/modal.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets(
'showModal builds a new route with specified barrier properties',
(WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Builder(builder: (BuildContext context) {
return Center(
child: ElevatedButton(
onPressed: () {
showModal<void>(
context: context,
configuration: _TestModalConfiguration(),
builder: (BuildContext context) {
return const _FlutterLogoModal();
},
);
},
child: const Icon(Icons.add),
),
);
}),
),
),
);
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
// New route containing _FlutterLogoModal is present.
expect(find.byType(_FlutterLogoModal), findsOneWidget);
final ModalBarrier topModalBarrier = tester.widget<ModalBarrier>(
find.byType(ModalBarrier).at(1),
);
// Verify new route's modal barrier properties are correct.
expect(topModalBarrier.color, Colors.green);
expect(topModalBarrier.barrierSemanticsDismissible, true);
expect(topModalBarrier.semanticsLabel, 'customLabel');
},
);
testWidgets(
'showModal forwards animation',
(WidgetTester tester) async {
final GlobalKey key = GlobalKey();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Builder(builder: (BuildContext context) {
return Center(
child: ElevatedButton(
onPressed: () {
showModal<void>(
context: context,
configuration: _TestModalConfiguration(),
builder: (BuildContext context) {
return _FlutterLogoModal(key: key);
},
);
},
child: const Icon(Icons.add),
),
);
}),
),
),
);
// Start forwards animation
await tester.tap(find.byType(ElevatedButton));
await tester.pump();
// Opacity duration: Linear transition throughout 300ms
double topFadeTransitionOpacity = _getOpacity(key, tester);
expect(topFadeTransitionOpacity, 0.0);
// Halfway through forwards animation.
await tester.pump(const Duration(milliseconds: 150));
topFadeTransitionOpacity = _getOpacity(key, tester);
expect(topFadeTransitionOpacity, 0.5);
// The end of the transition.
await tester.pump(const Duration(milliseconds: 150));
topFadeTransitionOpacity = _getOpacity(key, tester);
expect(topFadeTransitionOpacity, 1.0);
await tester.pump(const Duration(milliseconds: 1));
expect(find.byType(_FlutterLogoModal), findsOneWidget);
},
);
testWidgets(
'showModal reverse animation',
(WidgetTester tester) async {
final GlobalKey key = GlobalKey();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Builder(builder: (BuildContext context) {
return Center(
child: ElevatedButton(
onPressed: () {
showModal<void>(
context: context,
configuration: _TestModalConfiguration(),
builder: (BuildContext context) {
return _FlutterLogoModal(key: key);
},
);
},
child: const Icon(Icons.add),
),
);
}),
),
),
);
// Start forwards animation
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(find.byType(_FlutterLogoModal), findsOneWidget);
await tester.tapAt(Offset.zero);
await tester.pump();
// Opacity duration: Linear transition throughout 200ms
double topFadeTransitionOpacity = _getOpacity(key, tester);
expect(topFadeTransitionOpacity, 1.0);
// Halfway through forwards animation.
await tester.pump(const Duration(milliseconds: 100));
topFadeTransitionOpacity = _getOpacity(key, tester);
expect(topFadeTransitionOpacity, 0.5);
// The end of the transition.
await tester.pump(const Duration(milliseconds: 100));
topFadeTransitionOpacity = _getOpacity(key, tester);
expect(topFadeTransitionOpacity, 0.0);
await tester.pump(const Duration(milliseconds: 1));
expect(find.byType(_FlutterLogoModal), findsNothing);
},
);
testWidgets(
'showModal builds a new route with specified barrier properties '
'with default configuration(FadeScaleTransitionConfiguration)',
(WidgetTester tester) async {
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Builder(builder: (BuildContext context) {
return Center(
child: ElevatedButton(
onPressed: () {
showModal<void>(
context: context,
builder: (BuildContext context) {
return const _FlutterLogoModal();
},
);
},
child: const Icon(Icons.add),
),
);
}),
),
),
);
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
// New route containing _FlutterLogoModal is present.
expect(find.byType(_FlutterLogoModal), findsOneWidget);
final ModalBarrier topModalBarrier = tester.widget<ModalBarrier>(
find.byType(ModalBarrier).at(1),
);
// Verify new route's modal barrier properties are correct.
expect(topModalBarrier.color, Colors.black54);
expect(topModalBarrier.barrierSemanticsDismissible, true);
expect(topModalBarrier.semanticsLabel, 'Dismiss');
},
);
testWidgets(
'showModal forwards animation '
'with default configuration(FadeScaleTransitionConfiguration)',
(WidgetTester tester) async {
final GlobalKey key = GlobalKey();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Builder(builder: (BuildContext context) {
return Center(
child: ElevatedButton(
onPressed: () {
showModal<void>(
context: context,
builder: (BuildContext context) {
return _FlutterLogoModal(key: key);
},
);
},
child: const Icon(Icons.add),
),
);
}),
),
),
);
// Start forwards animation
await tester.tap(find.byType(ElevatedButton));
await tester.pump();
// Opacity duration: First 30% of 150ms, linear transition
double topFadeTransitionOpacity = _getOpacity(key, tester);
double topScale = _getScale(key, tester);
expect(topFadeTransitionOpacity, 0.0);
expect(topScale, 0.80);
// 3/10 * 150ms = 45ms (total opacity animation duration)
// 1/2 * 45ms = ~23ms elapsed for halfway point of opacity
// animation
await tester.pump(const Duration(milliseconds: 23));
topFadeTransitionOpacity = _getOpacity(key, tester);
expect(topFadeTransitionOpacity, closeTo(0.5, 0.05));
topScale = _getScale(key, tester);
expect(topScale, greaterThan(0.80));
expect(topScale, lessThan(1.0));
// End of opacity animation.
await tester.pump(const Duration(milliseconds: 22));
topFadeTransitionOpacity = _getOpacity(key, tester);
expect(topFadeTransitionOpacity, 1.0);
topScale = _getScale(key, tester);
expect(topScale, greaterThan(0.80));
expect(topScale, lessThan(1.0));
// 100ms into the animation
await tester.pump(const Duration(milliseconds: 55));
topScale = _getScale(key, tester);
expect(topScale, greaterThan(0.80));
expect(topScale, lessThan(1.0));
// Get to the end of the animation
await tester.pump(const Duration(milliseconds: 50));
topScale = _getScale(key, tester);
expect(topScale, 1.0);
await tester.pump(const Duration(milliseconds: 1));
expect(find.byType(_FlutterLogoModal), findsOneWidget);
},
);
testWidgets(
'showModal reverse animation '
'with default configuration(FadeScaleTransitionConfiguration)',
(WidgetTester tester) async {
final GlobalKey key = GlobalKey();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Builder(builder: (BuildContext context) {
return Center(
child: ElevatedButton(
onPressed: () {
showModal<void>(
context: context,
builder: (BuildContext context) {
return _FlutterLogoModal(key: key);
},
);
},
child: const Icon(Icons.add),
),
);
}),
),
),
);
// Start forwards animation
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
expect(find.byType(_FlutterLogoModal), findsOneWidget);
// Tap on modal barrier to start reverse animation.
await tester.tapAt(Offset.zero);
await tester.pump();
// Opacity duration: Linear transition throughout 75ms
// No scale animations on exit transition.
double topFadeTransitionOpacity = _getOpacity(key, tester);
double topScale = _getScale(key, tester);
expect(topFadeTransitionOpacity, 1.0);
expect(topScale, 1.0);
await tester.pump(const Duration(milliseconds: 25));
topFadeTransitionOpacity = _getOpacity(key, tester);
topScale = _getScale(key, tester);
expect(topFadeTransitionOpacity, closeTo(0.66, 0.05));
expect(topScale, 1.0);
await tester.pump(const Duration(milliseconds: 25));
topFadeTransitionOpacity = _getOpacity(key, tester);
topScale = _getScale(key, tester);
expect(topFadeTransitionOpacity, closeTo(0.33, 0.05));
expect(topScale, 1.0);
// End of opacity animation
await tester.pump(const Duration(milliseconds: 25));
topFadeTransitionOpacity = _getOpacity(key, tester);
expect(topFadeTransitionOpacity, 0.0);
topScale = _getScale(key, tester);
expect(topScale, 1.0);
await tester.pump(const Duration(milliseconds: 1));
expect(find.byType(_FlutterLogoModal), findsNothing);
},
);
testWidgets(
'State is not lost when transitioning',
(WidgetTester tester) async {
final GlobalKey bottomKey = GlobalKey();
final GlobalKey topKey = GlobalKey();
await tester.pumpWidget(
MaterialApp(
home: Scaffold(
body: Builder(builder: (BuildContext context) {
return Center(
child: Column(
children: <Widget>[
ElevatedButton(
onPressed: () {
showModal<void>(
context: context,
configuration: _TestModalConfiguration(),
builder: (BuildContext context) {
return _FlutterLogoModal(
key: topKey,
name: 'top route',
);
},
);
},
child: const Icon(Icons.add),
),
_FlutterLogoModal(
key: bottomKey,
name: 'bottom route',
),
],
),
);
}),
),
),
);
// The bottom route's state should already exist.
final _FlutterLogoModalState bottomState = tester.state(
find.byKey(bottomKey),
);
expect(bottomState.widget.name, 'bottom route');
// Start the enter transition of the modal route.
await tester.tap(find.byType(ElevatedButton));
await tester.pump();
await tester.pump();
// The bottom route's state should be retained at the start of the
// transition.
expect(
tester.state(find.byKey(bottomKey)),
bottomState,
);
// The top route's state should be created.
final _FlutterLogoModalState topState = tester.state(
find.byKey(topKey),
);
expect(topState.widget.name, 'top route');
// Halfway point of forwards animation.
await tester.pump(const Duration(milliseconds: 150));
expect(
tester.state(find.byKey(bottomKey)),
bottomState,
);
expect(
tester.state(find.byKey(topKey)),
topState,
);
// End the transition and see if top and bottom routes'
// states persist.
await tester.pumpAndSettle();
expect(
tester.state(find.byKey(
bottomKey,
skipOffstage: false,
)),
bottomState,
);
expect(
tester.state(find.byKey(topKey)),
topState,
);
// Start the reverse animation. Both top and bottom
// routes' states should persist.
await tester.tapAt(Offset.zero);
await tester.pump();
expect(
tester.state(find.byKey(bottomKey)),
bottomState,
);
expect(
tester.state(find.byKey(topKey)),
topState,
);
// Halfway point of the exit transition.
await tester.pump(const Duration(milliseconds: 100));
expect(
tester.state(find.byKey(bottomKey)),
bottomState,
);
expect(
tester.state(find.byKey(topKey)),
topState,
);
// End the exit transition. The bottom route's state should
// persist, whereas the top route's state should no longer
// be present.
await tester.pumpAndSettle();
expect(
tester.state(find.byKey(bottomKey)),
bottomState,
);
expect(find.byKey(topKey), findsNothing);
},
);
testWidgets(
'showModal builds a new route with specified route settings',
(WidgetTester tester) async {
const RouteSettings routeSettings = RouteSettings(
name: 'route-name',
arguments: 'arguments',
);
final Widget button = Builder(builder: (BuildContext context) {
return Center(
child: ElevatedButton(
onPressed: () {
showModal<void>(
context: context,
configuration: _TestModalConfiguration(),
routeSettings: routeSettings,
builder: (BuildContext context) {
return const _FlutterLogoModal();
},
);
},
child: const Icon(Icons.add),
),
);
});
await tester.pumpWidget(_boilerplate(button));
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
// New route containing _FlutterLogoModal is present.
expect(find.byType(_FlutterLogoModal), findsOneWidget);
// Expect the last route pushed to the navigator to contain RouteSettings
// equal to the RouteSettings passed to showModal
final ModalRoute<dynamic> modalRoute = ModalRoute.of(
tester.element(find.byType(_FlutterLogoModal)),
)!;
expect(modalRoute.settings, routeSettings);
},
);
testWidgets(
'showModal builds a new route with specified image filter',
(WidgetTester tester) async {
final ui.ImageFilter filter = ui.ImageFilter.blur(sigmaX: 1, sigmaY: 1);
final Widget button = Builder(builder: (BuildContext context) {
return Center(
child: ElevatedButton(
onPressed: () {
showModal<void>(
context: context,
configuration: _TestModalConfiguration(),
filter: filter,
builder: (BuildContext context) {
return const _FlutterLogoModal();
},
);
},
child: const Icon(Icons.add),
),
);
});
await tester.pumpWidget(_boilerplate(button));
await tester.tap(find.byType(ElevatedButton));
await tester.pumpAndSettle();
// New route containing _FlutterLogoModal is present.
expect(find.byType(_FlutterLogoModal), findsOneWidget);
final BackdropFilter backdropFilter = tester.widget<BackdropFilter>(
find.byType(BackdropFilter),
);
// Verify new route's backdrop filter has been applied
expect(backdropFilter.filter, filter);
},
);
}
Widget _boilerplate(Widget child) => MaterialApp(home: Scaffold(body: child));
double _getOpacity(GlobalKey key, WidgetTester tester) {
final Finder finder = find.ancestor(
of: find.byKey(key),
matching: find.byType(FadeTransition),
);
return tester.widgetList(finder).fold<double>(1.0, (double a, Widget widget) {
final FadeTransition transition = widget as FadeTransition;
return a * transition.opacity.value;
});
}
double _getScale(GlobalKey key, WidgetTester tester) {
final Finder finder = find.ancestor(
of: find.byKey(key),
matching: find.byType(ScaleTransition),
);
return tester.widgetList(finder).fold<double>(1.0, (double a, Widget widget) {
final ScaleTransition transition = widget as ScaleTransition;
return a * transition.scale.value;
});
}
class _FlutterLogoModal extends StatefulWidget {
const _FlutterLogoModal({
super.key,
this.name,
});
final String? name;
@override
_FlutterLogoModalState createState() => _FlutterLogoModalState();
}
class _FlutterLogoModalState extends State<_FlutterLogoModal> {
@override
Widget build(BuildContext context) {
return const Center(
child: SizedBox(
width: 250,
height: 250,
child: Material(
child: Center(
child: FlutterLogo(size: 250),
),
),
),
);
}
}
class _TestModalConfiguration extends ModalConfiguration {
_TestModalConfiguration()
: super(
barrierColor: Colors.green,
barrierDismissible: true,
barrierLabel: 'customLabel',
transitionDuration: const Duration(milliseconds: 300),
reverseTransitionDuration: const Duration(milliseconds: 200),
);
@override
Widget transitionBuilder(
BuildContext context,
Animation<double> animation,
Animation<double> secondaryAnimation,
Widget child,
) {
return FadeTransition(
opacity: animation,
child: child,
);
}
}
| packages/packages/animations/test/modal_test.dart/0 | {
"file_path": "packages/packages/animations/test/modal_test.dart",
"repo_id": "packages",
"token_count": 9177
} | 895 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.camera;
/**
* These are the states that the camera can be in. The camera can only take one photo at a time so
* this state describes the state of the camera itself. The camera works like a pipeline where we
* feed it requests through. It can only process one tasks at a time.
*/
public enum CameraState {
/** Idle, showing preview and not capturing anything. */
STATE_PREVIEW,
/** Starting and waiting for autofocus to complete. */
STATE_WAITING_FOCUS,
/** Start performing autoexposure. */
STATE_WAITING_PRECAPTURE_START,
/** waiting for autoexposure to complete. */
STATE_WAITING_PRECAPTURE_DONE,
/** Capturing an image. */
STATE_CAPTURING,
}
| packages/packages/camera/camera_android/android/src/main/java/io/flutter/plugins/camera/CameraState.java/0 | {
"file_path": "packages/packages/camera/camera_android/android/src/main/java/io/flutter/plugins/camera/CameraState.java",
"repo_id": "packages",
"token_count": 245
} | 896 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
//
// Note: the code in this file is taken directly from the official Google MLKit example:
// https://github.com/googlesamples/mlkit
package io.flutter.plugins.camera.media;
import android.media.Image;
import androidx.annotation.NonNull;
import java.nio.ByteBuffer;
public class ImageStreamReaderUtils {
/**
* Converts YUV_420_888 to NV21 bytebuffer.
*
* <p>The NV21 format consists of a single byte array containing the Y, U and V values. For an
* image of size S, the first S positions of the array contain all the Y values. The remaining
* positions contain interleaved V and U values. U and V are subsampled by a factor of 2 in both
* dimensions, so there are S/4 U values and S/4 V values. In summary, the NV21 array will contain
* S Y values followed by S/4 VU values: YYYYYYYYYYYYYY(...)YVUVUVUVU(...)VU
*
* <p>YUV_420_888 is a generic format that can describe any YUV image where U and V are subsampled
* by a factor of 2 in both dimensions. {@link Image#getPlanes} returns an array with the Y, U and
* V planes. The Y plane is guaranteed not to be interleaved, so we can just copy its values into
* the first part of the NV21 array. The U and V planes may already have the representation in the
* NV21 format. This happens if the planes share the same buffer, the V buffer is one position
* before the U buffer and the planes have a pixelStride of 2. If this is case, we can just copy
* them to the NV21 array.
*
* <p>https://github.com/googlesamples/mlkit/blob/master/android/vision-quickstart/app/src/main/java/com/google/mlkit/vision/demo/BitmapUtils.java
*/
@NonNull
public ByteBuffer yuv420ThreePlanesToNV21(
@NonNull Image.Plane[] yuv420888planes, int width, int height) {
int imageSize = width * height;
byte[] out = new byte[imageSize + 2 * (imageSize / 4)];
if (areUVPlanesNV21(yuv420888planes, width, height)) {
// Copy the Y values.
yuv420888planes[0].getBuffer().get(out, 0, imageSize);
ByteBuffer uBuffer = yuv420888planes[1].getBuffer();
ByteBuffer vBuffer = yuv420888planes[2].getBuffer();
// Get the first V value from the V buffer, since the U buffer does not contain it.
vBuffer.get(out, imageSize, 1);
// Copy the first U value and the remaining VU values from the U buffer.
uBuffer.get(out, imageSize + 1, 2 * imageSize / 4 - 1);
} else {
// Fallback to copying the UV values one by one, which is slower but also works.
// Unpack Y.
unpackPlane(yuv420888planes[0], width, height, out, 0, 1);
// Unpack U.
unpackPlane(yuv420888planes[1], width, height, out, imageSize + 1, 2);
// Unpack V.
unpackPlane(yuv420888planes[2], width, height, out, imageSize, 2);
}
return ByteBuffer.wrap(out);
}
/**
* Copyright 2020 Google LLC. All rights reserved.
*
* <p>Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file
* except in compliance with the License. You may obtain a copy of the License at
*
* <p>http://www.apache.org/licenses/LICENSE-2.0
*
* <p>Unless required by applicable law or agreed to in writing, software distributed under the
* License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language governing permissions and
* limitations under the License.
*
* <p>Checks if the UV plane buffers of a YUV_420_888 image are in the NV21 format.
*
* <p>https://github.com/googlesamples/mlkit/blob/master/android/vision-quickstart/app/src/main/java/com/google/mlkit/vision/demo/BitmapUtils.java
*/
private static boolean areUVPlanesNV21(@NonNull Image.Plane[] planes, int width, int height) {
int imageSize = width * height;
ByteBuffer uBuffer = planes[1].getBuffer();
ByteBuffer vBuffer = planes[2].getBuffer();
// Backup buffer properties.
int vBufferPosition = vBuffer.position();
int uBufferLimit = uBuffer.limit();
// Advance the V buffer by 1 byte, since the U buffer will not contain the first V value.
vBuffer.position(vBufferPosition + 1);
// Chop off the last byte of the U buffer, since the V buffer will not contain the last U value.
uBuffer.limit(uBufferLimit - 1);
// Check that the buffers are equal and have the expected number of elements.
boolean areNV21 =
(vBuffer.remaining() == (2 * imageSize / 4 - 2)) && (vBuffer.compareTo(uBuffer) == 0);
// Restore buffers to their initial state.
vBuffer.position(vBufferPosition);
uBuffer.limit(uBufferLimit);
return areNV21;
}
/**
* Copyright 2020 Google LLC. All rights reserved.
*
* <p>Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file
* except in compliance with the License. You may obtain a copy of the License at
*
* <p>http://www.apache.org/licenses/LICENSE-2.0
*
* <p>Unless required by applicable law or agreed to in writing, software distributed under the
* License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language governing permissions and
* limitations under the License.
*
* <p>Unpack an image plane into a byte array.
*
* <p>The input plane data will be copied in 'out', starting at 'offset' and every pixel will be
* spaced by 'pixelStride'. Note that there is no row padding on the output.
*
* <p>https://github.com/googlesamples/mlkit/blob/master/android/vision-quickstart/app/src/main/java/com/google/mlkit/vision/demo/BitmapUtils.java
*/
private static void unpackPlane(
@NonNull Image.Plane plane, int width, int height, byte[] out, int offset, int pixelStride)
throws IllegalStateException {
ByteBuffer buffer = plane.getBuffer();
buffer.rewind();
// Compute the size of the current plane.
// We assume that it has the aspect ratio as the original image.
int numRow = (buffer.limit() + plane.getRowStride() - 1) / plane.getRowStride();
if (numRow == 0) {
return;
}
int scaleFactor = height / numRow;
int numCol = width / scaleFactor;
// Extract the data in the output buffer.
int outputPos = offset;
int rowStart = 0;
for (int row = 0; row < numRow; row++) {
int inputPos = rowStart;
for (int col = 0; col < numCol; col++) {
out[outputPos] = buffer.get(inputPos);
outputPos += pixelStride;
inputPos += plane.getPixelStride();
}
rowStart += plane.getRowStride();
}
}
}
| packages/packages/camera/camera_android/android/src/main/java/io/flutter/plugins/camera/media/ImageStreamReaderUtils.java/0 | {
"file_path": "packages/packages/camera/camera_android/android/src/main/java/io/flutter/plugins/camera/media/ImageStreamReaderUtils.java",
"repo_id": "packages",
"token_count": 2288
} | 897 |
# Contributing to camera\_android\_camerax
## Plugin structure
The `camera_platform_interface` implementation is located at
`lib/src/android_camera_camerax.dart`, and it is implemented using Dart classes
that are wrapped versions of native Android Java classes.
In this approach, each native Android library used in the plugin implementation
is represented by an equivalent Dart class. Instances of these classes are
considered paired and represent each other in Java and Dart, respectively. An
`InstanceManager`, which is essentially a map between `long` identifiers and
objects that also provides notifications when an object has become unreachable
by memory. There is both a Dart and Java `InstanceManager` implementation, so
when a Dart instance is created that represens an Android native instance,
both are stored in the `InstanceManager` of their respective language with a
shared `long` identifier. These `InstanceManager`s take callbacks that run
when objects become unrechable or removed, allowing the Dart library to easily
handle removing references to native resources automatically. To ensure all
created instances are properly managed and to more easily allow for testing,
each wrapped Android native class in Dart takes an `InstanceManager` and has
a detached constructor, a constructor that allows for the creation of instances
not attached to the `InstanceManager` and unlinked to a paired Android native
instance.
In `lib/src/`, you will find all of the Dart-wrapped native Android classes that
the plugin currently uses in its implementation. As aforementioned, each of
these classes uses an `InstanceManager` (implementation in `instance_manager.dart`)
to manage objects that are created by the plugin implementation that map to objects
of the same type created in the native Android code. This plugin uses [`pigeon`][1]
to generate the communication layer between Flutter and native Android code, so each
of these Dart-wrapped classes may also have Host API and Flutter API implementations
that handle communication to the host native Android platform and from the host
native Android platform, respectively. The communication interface is defined in
the `pigeons/camerax_library.dart` file. After editing the communication interface,
regenerate the communication layer by running
`dart run pigeon --input pigeons/camerax_library.dart` from the plugin root.
In the native Java Android code in `android/src/main/java/io/flutter/plugins/camerax/`,
you'll find the Host API and Flutter API implementations of the same classes
wrapped with Dart in `lib/src/` that handle communication from that Dart code
and to that Dart code, respectively. The Host API implementations should directly
delegate calls to the CameraX or other wrapped Android libraries and should not
have any additional logic or abstraction; any exceptions should be thoroughly
documented in the code. As aforementioned, the objects created in the native
Android code map to objects created on the Dart side and are also managed by
an `InstanceManager` (implementation in `InstanceManager.java`).
If CameraX or other Android classes that you need to access do not have a
duplicately named implementation in `lib/src/`, then follow the same structure
described above to add them. Please note that any Dart-wrapped native Android
classes that you add should extend `JavaObject`. Additionally, they should be
annotated as `@immutable` to avoid lint errors with mock objects that are
generated for them that you may use for testing.
For more information, please see the [design document][2] or feel free
to ask any questions on the #hackers-ecosystem channel on [Discord][6]. For
more information on contributing packages in general, check out our
[contribution guide][3].
## Testing
While none of the generated `pigeon` files are tested, all plugin impelementation and
wrapped native Android classes (Java & Dart) are tested. You can find the Java tests under
`android/src/test/java/io/flutter/plugins/camerax/` and the Dart tests under `test/`. To
run these tests, please see the instructions in the [running plugin tests guide][5].
Besides [`pigeon`][1], this plugin also uses [`mockito`][4] to generate mock objects for
testing purposes. To generate the mock objects, run
`dart run build_runner build --delete-conflicting-outputs`.
[1]: https://pub.dev/packages/pigeon
[2]: https://docs.google.com/document/d/1wXB1zNzYhd2SxCu1_BK3qmNWRhonTB6qdv4erdtBQqo/edit?usp=sharing&resourcekey=0-WOBqqOKiO9SARnziBg28pg
[3]: https://github.com/flutter/packages/blob/main/CONTRIBUTING.md
[4]: https://pub.dev/packages/mockito
[5]: https://github.com/flutter/flutter/wiki/Plugin-Tests#running-tests
[6]: https://github.com/flutter/flutter/wiki/Chat | packages/packages/camera/camera_android_camerax/CONTRIBUTING.md/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/CONTRIBUTING.md",
"repo_id": "packages",
"token_count": 1177
} | 898 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.camerax;
import androidx.annotation.NonNull;
import androidx.annotation.VisibleForTesting;
import androidx.camera.core.CameraInfo;
import androidx.camera.core.CameraState;
import androidx.camera.core.ExposureState;
import androidx.camera.core.ZoomState;
import androidx.lifecycle.LiveData;
import io.flutter.plugin.common.BinaryMessenger;
import io.flutter.plugins.camerax.GeneratedCameraXLibrary.CameraInfoHostApi;
import io.flutter.plugins.camerax.GeneratedCameraXLibrary.LiveDataSupportedType;
import java.util.Objects;
public class CameraInfoHostApiImpl implements CameraInfoHostApi {
private final BinaryMessenger binaryMessenger;
private final InstanceManager instanceManager;
@VisibleForTesting public @NonNull LiveDataFlutterApiWrapper liveDataFlutterApiWrapper;
public CameraInfoHostApiImpl(
@NonNull BinaryMessenger binaryMessenger, @NonNull InstanceManager instanceManager) {
this.binaryMessenger = binaryMessenger;
this.instanceManager = instanceManager;
this.liveDataFlutterApiWrapper =
new LiveDataFlutterApiWrapper(binaryMessenger, instanceManager);
}
/**
* Retrieves the sensor rotation degrees of the {@link androidx.camera.core.Camera} that is
* represented by the {@link CameraInfo} with the specified identifier.
*/
@Override
@NonNull
public Long getSensorRotationDegrees(@NonNull Long identifier) {
CameraInfo cameraInfo =
(CameraInfo) Objects.requireNonNull(instanceManager.getInstance(identifier));
return Long.valueOf(cameraInfo.getSensorRotationDegrees());
}
/**
* Retrieves the {@link LiveData} of the {@link CameraState} that is tied to the {@link
* androidx.camera.core.Camera} that is represented by the {@link CameraInfo} with the specified
* identifier.
*/
@Override
@NonNull
public Long getCameraState(@NonNull Long identifier) {
CameraInfo cameraInfo =
(CameraInfo) Objects.requireNonNull(instanceManager.getInstance(identifier));
LiveData<CameraState> liveCameraState = cameraInfo.getCameraState();
liveDataFlutterApiWrapper.create(
liveCameraState, LiveDataSupportedType.CAMERA_STATE, reply -> {});
return instanceManager.getIdentifierForStrongReference(liveCameraState);
}
/**
* Retrieves the {@link ExposureState} of the {@link CameraInfo} with the specified identifier.
*/
@Override
@NonNull
public Long getExposureState(@NonNull Long identifier) {
CameraInfo cameraInfo =
(CameraInfo) Objects.requireNonNull(instanceManager.getInstance(identifier));
ExposureState exposureState = cameraInfo.getExposureState();
ExposureStateFlutterApiImpl exposureStateFlutterApiImpl =
new ExposureStateFlutterApiImpl(binaryMessenger, instanceManager);
exposureStateFlutterApiImpl.create(exposureState, result -> {});
return instanceManager.getIdentifierForStrongReference(exposureState);
}
/**
* Retrieves the {@link LiveData} of the {@link ZoomState} of the {@link CameraInfo} with the
* specified identifier.
*/
@NonNull
@Override
public Long getZoomState(@NonNull Long identifier) {
CameraInfo cameraInfo =
(CameraInfo) Objects.requireNonNull(instanceManager.getInstance(identifier));
LiveData<ZoomState> zoomState = cameraInfo.getZoomState();
LiveDataFlutterApiWrapper liveDataFlutterApiWrapper =
new LiveDataFlutterApiWrapper(binaryMessenger, instanceManager);
liveDataFlutterApiWrapper.create(zoomState, LiveDataSupportedType.ZOOM_STATE, reply -> {});
return instanceManager.getIdentifierForStrongReference(zoomState);
}
}
| packages/packages/camera/camera_android_camerax/android/src/main/java/io/flutter/plugins/camerax/CameraInfoHostApiImpl.java/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/android/src/main/java/io/flutter/plugins/camerax/CameraInfoHostApiImpl.java",
"repo_id": "packages",
"token_count": 1173
} | 899 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// Autogenerated from Pigeon (v9.2.5), do not edit directly.
// See also: https://pub.dev/packages/pigeon
package io.flutter.plugins.camerax;
import android.util.Log;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import io.flutter.plugin.common.BasicMessageChannel;
import io.flutter.plugin.common.BinaryMessenger;
import io.flutter.plugin.common.MessageCodec;
import io.flutter.plugin.common.StandardMessageCodec;
import java.io.ByteArrayOutputStream;
import java.nio.ByteBuffer;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Map;
/** Generated class from Pigeon. */
@SuppressWarnings({"unused", "unchecked", "CodeBlock2Expr", "RedundantSuppression", "serial"})
public class GeneratedCameraXLibrary {
/** Error class for passing custom error details to Flutter via a thrown PlatformException. */
public static class FlutterError extends RuntimeException {
/** The error code. */
public final String code;
/** The error details. Must be a datatype supported by the api codec. */
public final Object details;
public FlutterError(@NonNull String code, @Nullable String message, @Nullable Object details) {
super(message);
this.code = code;
this.details = details;
}
}
@NonNull
protected static ArrayList<Object> wrapError(@NonNull Throwable exception) {
ArrayList<Object> errorList = new ArrayList<Object>(3);
if (exception instanceof FlutterError) {
FlutterError error = (FlutterError) exception;
errorList.add(error.code);
errorList.add(error.getMessage());
errorList.add(error.details);
} else {
errorList.add(exception.toString());
errorList.add(exception.getClass().getSimpleName());
errorList.add(
"Cause: " + exception.getCause() + ", Stacktrace: " + Log.getStackTraceString(exception));
}
return errorList;
}
/**
* The states the camera can be in.
*
* <p>See https://developer.android.com/reference/androidx/camera/core/CameraState.Type.
*/
public enum CameraStateType {
CLOSED(0),
CLOSING(1),
OPEN(2),
OPENING(3),
PENDING_OPEN(4);
final int index;
private CameraStateType(final int index) {
this.index = index;
}
}
/**
* The types (T) properly wrapped to be used as a LiveData<T>.
*
* <p>If you need to add another type to support a type S to use a LiveData<S> in this plugin,
* ensure the following is done on the Dart side:
*
* <p>* In `camera_android_camerax/lib/src/live_data.dart`, add new cases for S in
* `_LiveDataHostApiImpl#getValueFromInstances` to get the current value of type S from a
* LiveData<S> instance and in `LiveDataFlutterApiImpl#create` to create the expected type of
* LiveData<S> when requested.
*
* <p>On the native side, ensure the following is done:
*
* <p>* Make sure `LiveDataHostApiImpl#getValue` is updated to properly return identifiers for
* instances of type S. * Update `ObserverFlutterApiWrapper#onChanged` to properly handle
* receiving calls with instances of type S if a LiveData<S> instance is observed.
*/
public enum LiveDataSupportedType {
CAMERA_STATE(0),
ZOOM_STATE(1);
final int index;
private LiveDataSupportedType(final int index) {
this.index = index;
}
}
/**
* Video quality constraints that will be used by a QualitySelector to choose an appropriate video
* resolution.
*
* <p>These are pre-defined quality constants that are universally used for video.
*
* <p>See https://developer.android.com/reference/androidx/camera/video/Quality.
*/
public enum VideoQuality {
SD(0),
HD(1),
FHD(2),
UHD(3),
LOWEST(4),
HIGHEST(5);
final int index;
private VideoQuality(final int index) {
this.index = index;
}
}
/**
* Fallback rules for selecting video resolution.
*
* <p>See https://developer.android.com/reference/androidx/camera/video/FallbackStrategy.
*/
public enum VideoResolutionFallbackRule {
HIGHER_QUALITY_OR_LOWER_THAN(0),
HIGHER_QUALITY_THAN(1),
LOWER_QUALITY_OR_HIGHER_THAN(2),
LOWER_QUALITY_THAN(3);
final int index;
private VideoResolutionFallbackRule(final int index) {
this.index = index;
}
}
/**
* The types of capture request options this plugin currently supports.
*
* <p>If you need to add another option to support, ensure the following is done on the Dart side:
*
* <p>* In `camera_android_camerax/lib/src/capture_request_options.dart`, add new cases for this
* option in `_CaptureRequestOptionsHostApiImpl#createFromInstances` to create the expected Map
* entry of option key index and value to send to the native side.
*
* <p>On the native side, ensure the following is done:
*
* <p>* Update `CaptureRequestOptionsHostApiImpl#create` to set the correct `CaptureRequest` key
* with a valid value type for this option.
*
* <p>See https://developer.android.com/reference/android/hardware/camera2/CaptureRequest for the
* sorts of capture request options that can be supported via CameraX's interoperability with
* Camera2.
*/
public enum CaptureRequestKeySupportedType {
CONTROL_AE_LOCK(0);
final int index;
private CaptureRequestKeySupportedType(final int index) {
this.index = index;
}
}
/** Generated class from Pigeon that represents data sent in messages. */
public static final class ResolutionInfo {
private @NonNull Long width;
public @NonNull Long getWidth() {
return width;
}
public void setWidth(@NonNull Long setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"width\" is null.");
}
this.width = setterArg;
}
private @NonNull Long height;
public @NonNull Long getHeight() {
return height;
}
public void setHeight(@NonNull Long setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"height\" is null.");
}
this.height = setterArg;
}
/** Constructor is non-public to enforce null safety; use Builder. */
ResolutionInfo() {}
public static final class Builder {
private @Nullable Long width;
public @NonNull Builder setWidth(@NonNull Long setterArg) {
this.width = setterArg;
return this;
}
private @Nullable Long height;
public @NonNull Builder setHeight(@NonNull Long setterArg) {
this.height = setterArg;
return this;
}
public @NonNull ResolutionInfo build() {
ResolutionInfo pigeonReturn = new ResolutionInfo();
pigeonReturn.setWidth(width);
pigeonReturn.setHeight(height);
return pigeonReturn;
}
}
@NonNull
ArrayList<Object> toList() {
ArrayList<Object> toListResult = new ArrayList<Object>(2);
toListResult.add(width);
toListResult.add(height);
return toListResult;
}
static @NonNull ResolutionInfo fromList(@NonNull ArrayList<Object> list) {
ResolutionInfo pigeonResult = new ResolutionInfo();
Object width = list.get(0);
pigeonResult.setWidth(
(width == null) ? null : ((width instanceof Integer) ? (Integer) width : (Long) width));
Object height = list.get(1);
pigeonResult.setHeight(
(height == null)
? null
: ((height instanceof Integer) ? (Integer) height : (Long) height));
return pigeonResult;
}
}
/** Generated class from Pigeon that represents data sent in messages. */
public static final class CameraPermissionsErrorData {
private @NonNull String errorCode;
public @NonNull String getErrorCode() {
return errorCode;
}
public void setErrorCode(@NonNull String setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"errorCode\" is null.");
}
this.errorCode = setterArg;
}
private @NonNull String description;
public @NonNull String getDescription() {
return description;
}
public void setDescription(@NonNull String setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"description\" is null.");
}
this.description = setterArg;
}
/** Constructor is non-public to enforce null safety; use Builder. */
CameraPermissionsErrorData() {}
public static final class Builder {
private @Nullable String errorCode;
public @NonNull Builder setErrorCode(@NonNull String setterArg) {
this.errorCode = setterArg;
return this;
}
private @Nullable String description;
public @NonNull Builder setDescription(@NonNull String setterArg) {
this.description = setterArg;
return this;
}
public @NonNull CameraPermissionsErrorData build() {
CameraPermissionsErrorData pigeonReturn = new CameraPermissionsErrorData();
pigeonReturn.setErrorCode(errorCode);
pigeonReturn.setDescription(description);
return pigeonReturn;
}
}
@NonNull
ArrayList<Object> toList() {
ArrayList<Object> toListResult = new ArrayList<Object>(2);
toListResult.add(errorCode);
toListResult.add(description);
return toListResult;
}
static @NonNull CameraPermissionsErrorData fromList(@NonNull ArrayList<Object> list) {
CameraPermissionsErrorData pigeonResult = new CameraPermissionsErrorData();
Object errorCode = list.get(0);
pigeonResult.setErrorCode((String) errorCode);
Object description = list.get(1);
pigeonResult.setDescription((String) description);
return pigeonResult;
}
}
/** Generated class from Pigeon that represents data sent in messages. */
public static final class CameraStateTypeData {
private @NonNull CameraStateType value;
public @NonNull CameraStateType getValue() {
return value;
}
public void setValue(@NonNull CameraStateType setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"value\" is null.");
}
this.value = setterArg;
}
/** Constructor is non-public to enforce null safety; use Builder. */
CameraStateTypeData() {}
public static final class Builder {
private @Nullable CameraStateType value;
public @NonNull Builder setValue(@NonNull CameraStateType setterArg) {
this.value = setterArg;
return this;
}
public @NonNull CameraStateTypeData build() {
CameraStateTypeData pigeonReturn = new CameraStateTypeData();
pigeonReturn.setValue(value);
return pigeonReturn;
}
}
@NonNull
ArrayList<Object> toList() {
ArrayList<Object> toListResult = new ArrayList<Object>(1);
toListResult.add(value == null ? null : value.index);
return toListResult;
}
static @NonNull CameraStateTypeData fromList(@NonNull ArrayList<Object> list) {
CameraStateTypeData pigeonResult = new CameraStateTypeData();
Object value = list.get(0);
pigeonResult.setValue(value == null ? null : CameraStateType.values()[(int) value]);
return pigeonResult;
}
}
/** Generated class from Pigeon that represents data sent in messages. */
public static final class LiveDataSupportedTypeData {
private @NonNull LiveDataSupportedType value;
public @NonNull LiveDataSupportedType getValue() {
return value;
}
public void setValue(@NonNull LiveDataSupportedType setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"value\" is null.");
}
this.value = setterArg;
}
/** Constructor is non-public to enforce null safety; use Builder. */
LiveDataSupportedTypeData() {}
public static final class Builder {
private @Nullable LiveDataSupportedType value;
public @NonNull Builder setValue(@NonNull LiveDataSupportedType setterArg) {
this.value = setterArg;
return this;
}
public @NonNull LiveDataSupportedTypeData build() {
LiveDataSupportedTypeData pigeonReturn = new LiveDataSupportedTypeData();
pigeonReturn.setValue(value);
return pigeonReturn;
}
}
@NonNull
ArrayList<Object> toList() {
ArrayList<Object> toListResult = new ArrayList<Object>(1);
toListResult.add(value == null ? null : value.index);
return toListResult;
}
static @NonNull LiveDataSupportedTypeData fromList(@NonNull ArrayList<Object> list) {
LiveDataSupportedTypeData pigeonResult = new LiveDataSupportedTypeData();
Object value = list.get(0);
pigeonResult.setValue(value == null ? null : LiveDataSupportedType.values()[(int) value]);
return pigeonResult;
}
}
/** Generated class from Pigeon that represents data sent in messages. */
public static final class ExposureCompensationRange {
private @NonNull Long minCompensation;
public @NonNull Long getMinCompensation() {
return minCompensation;
}
public void setMinCompensation(@NonNull Long setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"minCompensation\" is null.");
}
this.minCompensation = setterArg;
}
private @NonNull Long maxCompensation;
public @NonNull Long getMaxCompensation() {
return maxCompensation;
}
public void setMaxCompensation(@NonNull Long setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"maxCompensation\" is null.");
}
this.maxCompensation = setterArg;
}
/** Constructor is non-public to enforce null safety; use Builder. */
ExposureCompensationRange() {}
public static final class Builder {
private @Nullable Long minCompensation;
public @NonNull Builder setMinCompensation(@NonNull Long setterArg) {
this.minCompensation = setterArg;
return this;
}
private @Nullable Long maxCompensation;
public @NonNull Builder setMaxCompensation(@NonNull Long setterArg) {
this.maxCompensation = setterArg;
return this;
}
public @NonNull ExposureCompensationRange build() {
ExposureCompensationRange pigeonReturn = new ExposureCompensationRange();
pigeonReturn.setMinCompensation(minCompensation);
pigeonReturn.setMaxCompensation(maxCompensation);
return pigeonReturn;
}
}
@NonNull
ArrayList<Object> toList() {
ArrayList<Object> toListResult = new ArrayList<Object>(2);
toListResult.add(minCompensation);
toListResult.add(maxCompensation);
return toListResult;
}
static @NonNull ExposureCompensationRange fromList(@NonNull ArrayList<Object> list) {
ExposureCompensationRange pigeonResult = new ExposureCompensationRange();
Object minCompensation = list.get(0);
pigeonResult.setMinCompensation(
(minCompensation == null)
? null
: ((minCompensation instanceof Integer)
? (Integer) minCompensation
: (Long) minCompensation));
Object maxCompensation = list.get(1);
pigeonResult.setMaxCompensation(
(maxCompensation == null)
? null
: ((maxCompensation instanceof Integer)
? (Integer) maxCompensation
: (Long) maxCompensation));
return pigeonResult;
}
}
/**
* Convenience class for sending lists of [Quality]s.
*
* <p>Generated class from Pigeon that represents data sent in messages.
*/
public static final class VideoQualityData {
private @NonNull VideoQuality quality;
public @NonNull VideoQuality getQuality() {
return quality;
}
public void setQuality(@NonNull VideoQuality setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"quality\" is null.");
}
this.quality = setterArg;
}
/** Constructor is non-public to enforce null safety; use Builder. */
VideoQualityData() {}
public static final class Builder {
private @Nullable VideoQuality quality;
public @NonNull Builder setQuality(@NonNull VideoQuality setterArg) {
this.quality = setterArg;
return this;
}
public @NonNull VideoQualityData build() {
VideoQualityData pigeonReturn = new VideoQualityData();
pigeonReturn.setQuality(quality);
return pigeonReturn;
}
}
@NonNull
ArrayList<Object> toList() {
ArrayList<Object> toListResult = new ArrayList<Object>(1);
toListResult.add(quality == null ? null : quality.index);
return toListResult;
}
static @NonNull VideoQualityData fromList(@NonNull ArrayList<Object> list) {
VideoQualityData pigeonResult = new VideoQualityData();
Object quality = list.get(0);
pigeonResult.setQuality(quality == null ? null : VideoQuality.values()[(int) quality]);
return pigeonResult;
}
}
/**
* Convenience class for building [FocusMeteringAction]s with multiple metering points.
*
* <p>Generated class from Pigeon that represents data sent in messages.
*/
public static final class MeteringPointInfo {
/** InstanceManager ID for a [MeteringPoint]. */
private @NonNull Long meteringPointId;
public @NonNull Long getMeteringPointId() {
return meteringPointId;
}
public void setMeteringPointId(@NonNull Long setterArg) {
if (setterArg == null) {
throw new IllegalStateException("Nonnull field \"meteringPointId\" is null.");
}
this.meteringPointId = setterArg;
}
/**
* The metering mode of the [MeteringPoint] whose ID is [meteringPointId].
*
* <p>Metering mode should be one of the [FocusMeteringAction] constants.
*/
private @Nullable Long meteringMode;
public @Nullable Long getMeteringMode() {
return meteringMode;
}
public void setMeteringMode(@Nullable Long setterArg) {
this.meteringMode = setterArg;
}
/** Constructor is non-public to enforce null safety; use Builder. */
MeteringPointInfo() {}
public static final class Builder {
private @Nullable Long meteringPointId;
public @NonNull Builder setMeteringPointId(@NonNull Long setterArg) {
this.meteringPointId = setterArg;
return this;
}
private @Nullable Long meteringMode;
public @NonNull Builder setMeteringMode(@Nullable Long setterArg) {
this.meteringMode = setterArg;
return this;
}
public @NonNull MeteringPointInfo build() {
MeteringPointInfo pigeonReturn = new MeteringPointInfo();
pigeonReturn.setMeteringPointId(meteringPointId);
pigeonReturn.setMeteringMode(meteringMode);
return pigeonReturn;
}
}
@NonNull
ArrayList<Object> toList() {
ArrayList<Object> toListResult = new ArrayList<Object>(2);
toListResult.add(meteringPointId);
toListResult.add(meteringMode);
return toListResult;
}
static @NonNull MeteringPointInfo fromList(@NonNull ArrayList<Object> list) {
MeteringPointInfo pigeonResult = new MeteringPointInfo();
Object meteringPointId = list.get(0);
pigeonResult.setMeteringPointId(
(meteringPointId == null)
? null
: ((meteringPointId instanceof Integer)
? (Integer) meteringPointId
: (Long) meteringPointId));
Object meteringMode = list.get(1);
pigeonResult.setMeteringMode(
(meteringMode == null)
? null
: ((meteringMode instanceof Integer) ? (Integer) meteringMode : (Long) meteringMode));
return pigeonResult;
}
}
public interface Result<T> {
@SuppressWarnings("UnknownNullness")
void success(T result);
void error(@NonNull Throwable error);
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface InstanceManagerHostApi {
/**
* Clear the native `InstanceManager`.
*
* <p>This is typically only used after a hot restart.
*/
void clear();
/** The codec used by InstanceManagerHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `InstanceManagerHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(
@NonNull BinaryMessenger binaryMessenger, @Nullable InstanceManagerHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.InstanceManagerHostApi.clear", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
try {
api.clear();
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface JavaObjectHostApi {
void dispose(@NonNull Long identifier);
/** The codec used by JavaObjectHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `JavaObjectHostApi` to handle messages through the `binaryMessenger`.
*/
static void setup(@NonNull BinaryMessenger binaryMessenger, @Nullable JavaObjectHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.JavaObjectHostApi.dispose", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
api.dispose((identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class JavaObjectFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public JavaObjectFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by JavaObjectFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void dispose(@NonNull Long identifierArg, @NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.JavaObjectFlutterApi.dispose", getCodec());
channel.send(
new ArrayList<Object>(Collections.singletonList(identifierArg)),
channelReply -> callback.reply(null));
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface CameraInfoHostApi {
@NonNull
Long getSensorRotationDegrees(@NonNull Long identifier);
@NonNull
Long getCameraState(@NonNull Long identifier);
@NonNull
Long getExposureState(@NonNull Long identifier);
@NonNull
Long getZoomState(@NonNull Long identifier);
/** The codec used by CameraInfoHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `CameraInfoHostApi` to handle messages through the `binaryMessenger`.
*/
static void setup(@NonNull BinaryMessenger binaryMessenger, @Nullable CameraInfoHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.CameraInfoHostApi.getSensorRotationDegrees",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
Long output =
api.getSensorRotationDegrees(
(identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.CameraInfoHostApi.getCameraState", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
Long output =
api.getCameraState(
(identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.CameraInfoHostApi.getExposureState",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
Long output =
api.getExposureState(
(identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.CameraInfoHostApi.getZoomState", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
Long output =
api.getZoomState((identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class CameraInfoFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public CameraInfoFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by CameraInfoFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void create(@NonNull Long identifierArg, @NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.CameraInfoFlutterApi.create", getCodec());
channel.send(
new ArrayList<Object>(Collections.singletonList(identifierArg)),
channelReply -> callback.reply(null));
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface CameraSelectorHostApi {
void create(@NonNull Long identifier, @Nullable Long lensFacing);
@NonNull
List<Long> filter(@NonNull Long identifier, @NonNull List<Long> cameraInfoIds);
/** The codec used by CameraSelectorHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `CameraSelectorHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(
@NonNull BinaryMessenger binaryMessenger, @Nullable CameraSelectorHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.CameraSelectorHostApi.create", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Number lensFacingArg = (Number) args.get(1);
try {
api.create(
(identifierArg == null) ? null : identifierArg.longValue(),
(lensFacingArg == null) ? null : lensFacingArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.CameraSelectorHostApi.filter", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
List<Long> cameraInfoIdsArg = (List<Long>) args.get(1);
try {
List<Long> output =
api.filter(
(identifierArg == null) ? null : identifierArg.longValue(),
cameraInfoIdsArg);
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class CameraSelectorFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public CameraSelectorFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by CameraSelectorFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void create(
@NonNull Long identifierArg, @Nullable Long lensFacingArg, @NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.CameraSelectorFlutterApi.create", getCodec());
channel.send(
new ArrayList<Object>(Arrays.asList(identifierArg, lensFacingArg)),
channelReply -> callback.reply(null));
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface ProcessCameraProviderHostApi {
void getInstance(@NonNull Result<Long> result);
@NonNull
List<Long> getAvailableCameraInfos(@NonNull Long identifier);
@NonNull
Long bindToLifecycle(
@NonNull Long identifier,
@NonNull Long cameraSelectorIdentifier,
@NonNull List<Long> useCaseIds);
@NonNull
Boolean isBound(@NonNull Long identifier, @NonNull Long useCaseIdentifier);
void unbind(@NonNull Long identifier, @NonNull List<Long> useCaseIds);
void unbindAll(@NonNull Long identifier);
/** The codec used by ProcessCameraProviderHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `ProcessCameraProviderHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(
@NonNull BinaryMessenger binaryMessenger, @Nullable ProcessCameraProviderHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.ProcessCameraProviderHostApi.getInstance",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
Result<Long> resultCallback =
new Result<Long>() {
public void success(Long result) {
wrapped.add(0, result);
reply.reply(wrapped);
}
public void error(Throwable error) {
ArrayList<Object> wrappedError = wrapError(error);
reply.reply(wrappedError);
}
};
api.getInstance(resultCallback);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.ProcessCameraProviderHostApi.getAvailableCameraInfos",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
List<Long> output =
api.getAvailableCameraInfos(
(identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.ProcessCameraProviderHostApi.bindToLifecycle",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Number cameraSelectorIdentifierArg = (Number) args.get(1);
List<Long> useCaseIdsArg = (List<Long>) args.get(2);
try {
Long output =
api.bindToLifecycle(
(identifierArg == null) ? null : identifierArg.longValue(),
(cameraSelectorIdentifierArg == null)
? null
: cameraSelectorIdentifierArg.longValue(),
useCaseIdsArg);
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.ProcessCameraProviderHostApi.isBound",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Number useCaseIdentifierArg = (Number) args.get(1);
try {
Boolean output =
api.isBound(
(identifierArg == null) ? null : identifierArg.longValue(),
(useCaseIdentifierArg == null) ? null : useCaseIdentifierArg.longValue());
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.ProcessCameraProviderHostApi.unbind",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
List<Long> useCaseIdsArg = (List<Long>) args.get(1);
try {
api.unbind(
(identifierArg == null) ? null : identifierArg.longValue(), useCaseIdsArg);
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.ProcessCameraProviderHostApi.unbindAll",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
api.unbindAll((identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class ProcessCameraProviderFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public ProcessCameraProviderFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by ProcessCameraProviderFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void create(@NonNull Long identifierArg, @NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.ProcessCameraProviderFlutterApi.create",
getCodec());
channel.send(
new ArrayList<Object>(Collections.singletonList(identifierArg)),
channelReply -> callback.reply(null));
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface CameraHostApi {
@NonNull
Long getCameraInfo(@NonNull Long identifier);
@NonNull
Long getCameraControl(@NonNull Long identifier);
/** The codec used by CameraHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/** Sets up an instance of `CameraHostApi` to handle messages through the `binaryMessenger`. */
static void setup(@NonNull BinaryMessenger binaryMessenger, @Nullable CameraHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.CameraHostApi.getCameraInfo", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
Long output =
api.getCameraInfo((identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.CameraHostApi.getCameraControl", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
Long output =
api.getCameraControl(
(identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class CameraFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public CameraFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by CameraFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void create(@NonNull Long identifierArg, @NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.CameraFlutterApi.create", getCodec());
channel.send(
new ArrayList<Object>(Collections.singletonList(identifierArg)),
channelReply -> callback.reply(null));
}
}
private static class SystemServicesHostApiCodec extends StandardMessageCodec {
public static final SystemServicesHostApiCodec INSTANCE = new SystemServicesHostApiCodec();
private SystemServicesHostApiCodec() {}
@Override
protected Object readValueOfType(byte type, @NonNull ByteBuffer buffer) {
switch (type) {
case (byte) 128:
return CameraPermissionsErrorData.fromList((ArrayList<Object>) readValue(buffer));
default:
return super.readValueOfType(type, buffer);
}
}
@Override
protected void writeValue(@NonNull ByteArrayOutputStream stream, Object value) {
if (value instanceof CameraPermissionsErrorData) {
stream.write(128);
writeValue(stream, ((CameraPermissionsErrorData) value).toList());
} else {
super.writeValue(stream, value);
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface SystemServicesHostApi {
void requestCameraPermissions(
@NonNull Boolean enableAudio, @NonNull Result<CameraPermissionsErrorData> result);
@NonNull
String getTempFilePath(@NonNull String prefix, @NonNull String suffix);
/** The codec used by SystemServicesHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return SystemServicesHostApiCodec.INSTANCE;
}
/**
* Sets up an instance of `SystemServicesHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(
@NonNull BinaryMessenger binaryMessenger, @Nullable SystemServicesHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.SystemServicesHostApi.requestCameraPermissions",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Boolean enableAudioArg = (Boolean) args.get(0);
Result<CameraPermissionsErrorData> resultCallback =
new Result<CameraPermissionsErrorData>() {
public void success(CameraPermissionsErrorData result) {
wrapped.add(0, result);
reply.reply(wrapped);
}
public void error(Throwable error) {
ArrayList<Object> wrappedError = wrapError(error);
reply.reply(wrappedError);
}
};
api.requestCameraPermissions(enableAudioArg, resultCallback);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.SystemServicesHostApi.getTempFilePath",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
String prefixArg = (String) args.get(0);
String suffixArg = (String) args.get(1);
try {
String output = api.getTempFilePath(prefixArg, suffixArg);
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class SystemServicesFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public SystemServicesFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by SystemServicesFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void onCameraError(@NonNull String errorDescriptionArg, @NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.SystemServicesFlutterApi.onCameraError",
getCodec());
channel.send(
new ArrayList<Object>(Collections.singletonList(errorDescriptionArg)),
channelReply -> callback.reply(null));
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface DeviceOrientationManagerHostApi {
void startListeningForDeviceOrientationChange(
@NonNull Boolean isFrontFacing, @NonNull Long sensorOrientation);
void stopListeningForDeviceOrientationChange();
@NonNull
Long getDefaultDisplayRotation();
/** The codec used by DeviceOrientationManagerHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `DeviceOrientationManagerHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(
@NonNull BinaryMessenger binaryMessenger, @Nullable DeviceOrientationManagerHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.DeviceOrientationManagerHostApi.startListeningForDeviceOrientationChange",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Boolean isFrontFacingArg = (Boolean) args.get(0);
Number sensorOrientationArg = (Number) args.get(1);
try {
api.startListeningForDeviceOrientationChange(
isFrontFacingArg,
(sensorOrientationArg == null) ? null : sensorOrientationArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.DeviceOrientationManagerHostApi.stopListeningForDeviceOrientationChange",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
try {
api.stopListeningForDeviceOrientationChange();
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.DeviceOrientationManagerHostApi.getDefaultDisplayRotation",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
try {
Long output = api.getDefaultDisplayRotation();
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class DeviceOrientationManagerFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public DeviceOrientationManagerFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by DeviceOrientationManagerFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void onDeviceOrientationChanged(
@NonNull String orientationArg, @NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.DeviceOrientationManagerFlutterApi.onDeviceOrientationChanged",
getCodec());
channel.send(
new ArrayList<Object>(Collections.singletonList(orientationArg)),
channelReply -> callback.reply(null));
}
}
private static class PreviewHostApiCodec extends StandardMessageCodec {
public static final PreviewHostApiCodec INSTANCE = new PreviewHostApiCodec();
private PreviewHostApiCodec() {}
@Override
protected Object readValueOfType(byte type, @NonNull ByteBuffer buffer) {
switch (type) {
case (byte) 128:
return ResolutionInfo.fromList((ArrayList<Object>) readValue(buffer));
default:
return super.readValueOfType(type, buffer);
}
}
@Override
protected void writeValue(@NonNull ByteArrayOutputStream stream, Object value) {
if (value instanceof ResolutionInfo) {
stream.write(128);
writeValue(stream, ((ResolutionInfo) value).toList());
} else {
super.writeValue(stream, value);
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface PreviewHostApi {
void create(
@NonNull Long identifier, @Nullable Long rotation, @Nullable Long resolutionSelectorId);
@NonNull
Long setSurfaceProvider(@NonNull Long identifier);
void releaseFlutterSurfaceTexture();
@NonNull
ResolutionInfo getResolutionInfo(@NonNull Long identifier);
void setTargetRotation(@NonNull Long identifier, @NonNull Long rotation);
/** The codec used by PreviewHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return PreviewHostApiCodec.INSTANCE;
}
/** Sets up an instance of `PreviewHostApi` to handle messages through the `binaryMessenger`. */
static void setup(@NonNull BinaryMessenger binaryMessenger, @Nullable PreviewHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.PreviewHostApi.create", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Number rotationArg = (Number) args.get(1);
Number resolutionSelectorIdArg = (Number) args.get(2);
try {
api.create(
(identifierArg == null) ? null : identifierArg.longValue(),
(rotationArg == null) ? null : rotationArg.longValue(),
(resolutionSelectorIdArg == null)
? null
: resolutionSelectorIdArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.PreviewHostApi.setSurfaceProvider",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
Long output =
api.setSurfaceProvider(
(identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.PreviewHostApi.releaseFlutterSurfaceTexture",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
try {
api.releaseFlutterSurfaceTexture();
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.PreviewHostApi.getResolutionInfo", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
ResolutionInfo output =
api.getResolutionInfo(
(identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.PreviewHostApi.setTargetRotation", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Number rotationArg = (Number) args.get(1);
try {
api.setTargetRotation(
(identifierArg == null) ? null : identifierArg.longValue(),
(rotationArg == null) ? null : rotationArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface VideoCaptureHostApi {
@NonNull
Long withOutput(@NonNull Long videoOutputId);
@NonNull
Long getOutput(@NonNull Long identifier);
void setTargetRotation(@NonNull Long identifier, @NonNull Long rotation);
/** The codec used by VideoCaptureHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `VideoCaptureHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(@NonNull BinaryMessenger binaryMessenger, @Nullable VideoCaptureHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.VideoCaptureHostApi.withOutput", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number videoOutputIdArg = (Number) args.get(0);
try {
Long output =
api.withOutput(
(videoOutputIdArg == null) ? null : videoOutputIdArg.longValue());
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.VideoCaptureHostApi.getOutput", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
Long output =
api.getOutput((identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.VideoCaptureHostApi.setTargetRotation",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Number rotationArg = (Number) args.get(1);
try {
api.setTargetRotation(
(identifierArg == null) ? null : identifierArg.longValue(),
(rotationArg == null) ? null : rotationArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class VideoCaptureFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public VideoCaptureFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by VideoCaptureFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void create(@NonNull Long identifierArg, @NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.VideoCaptureFlutterApi.create", getCodec());
channel.send(
new ArrayList<Object>(Collections.singletonList(identifierArg)),
channelReply -> callback.reply(null));
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface RecorderHostApi {
void create(
@NonNull Long identifier,
@Nullable Long aspectRatio,
@Nullable Long bitRate,
@Nullable Long qualitySelectorId);
@NonNull
Long getAspectRatio(@NonNull Long identifier);
@NonNull
Long getTargetVideoEncodingBitRate(@NonNull Long identifier);
@NonNull
Long prepareRecording(@NonNull Long identifier, @NonNull String path);
/** The codec used by RecorderHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `RecorderHostApi` to handle messages through the `binaryMessenger`.
*/
static void setup(@NonNull BinaryMessenger binaryMessenger, @Nullable RecorderHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.RecorderHostApi.create", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Number aspectRatioArg = (Number) args.get(1);
Number bitRateArg = (Number) args.get(2);
Number qualitySelectorIdArg = (Number) args.get(3);
try {
api.create(
(identifierArg == null) ? null : identifierArg.longValue(),
(aspectRatioArg == null) ? null : aspectRatioArg.longValue(),
(bitRateArg == null) ? null : bitRateArg.longValue(),
(qualitySelectorIdArg == null) ? null : qualitySelectorIdArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.RecorderHostApi.getAspectRatio", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
Long output =
api.getAspectRatio(
(identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.RecorderHostApi.getTargetVideoEncodingBitRate",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
Long output =
api.getTargetVideoEncodingBitRate(
(identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.RecorderHostApi.prepareRecording", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
String pathArg = (String) args.get(1);
try {
Long output =
api.prepareRecording(
(identifierArg == null) ? null : identifierArg.longValue(), pathArg);
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class RecorderFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public RecorderFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by RecorderFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void create(
@NonNull Long identifierArg,
@Nullable Long aspectRatioArg,
@Nullable Long bitRateArg,
@NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.RecorderFlutterApi.create", getCodec());
channel.send(
new ArrayList<Object>(Arrays.asList(identifierArg, aspectRatioArg, bitRateArg)),
channelReply -> callback.reply(null));
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface PendingRecordingHostApi {
@NonNull
Long start(@NonNull Long identifier);
/** The codec used by PendingRecordingHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `PendingRecordingHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(
@NonNull BinaryMessenger binaryMessenger, @Nullable PendingRecordingHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.PendingRecordingHostApi.start", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
Long output =
api.start((identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class PendingRecordingFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public PendingRecordingFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by PendingRecordingFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void create(@NonNull Long identifierArg, @NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.PendingRecordingFlutterApi.create", getCodec());
channel.send(
new ArrayList<Object>(Collections.singletonList(identifierArg)),
channelReply -> callback.reply(null));
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface RecordingHostApi {
void close(@NonNull Long identifier);
void pause(@NonNull Long identifier);
void resume(@NonNull Long identifier);
void stop(@NonNull Long identifier);
/** The codec used by RecordingHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `RecordingHostApi` to handle messages through the `binaryMessenger`.
*/
static void setup(@NonNull BinaryMessenger binaryMessenger, @Nullable RecordingHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.RecordingHostApi.close", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
api.close((identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.RecordingHostApi.pause", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
api.pause((identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.RecordingHostApi.resume", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
api.resume((identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.RecordingHostApi.stop", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
api.stop((identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class RecordingFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public RecordingFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by RecordingFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void create(@NonNull Long identifierArg, @NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.RecordingFlutterApi.create", getCodec());
channel.send(
new ArrayList<Object>(Collections.singletonList(identifierArg)),
channelReply -> callback.reply(null));
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface ImageCaptureHostApi {
void create(
@NonNull Long identifier,
@Nullable Long targetRotation,
@Nullable Long flashMode,
@Nullable Long resolutionSelectorId);
void setFlashMode(@NonNull Long identifier, @NonNull Long flashMode);
void takePicture(@NonNull Long identifier, @NonNull Result<String> result);
void setTargetRotation(@NonNull Long identifier, @NonNull Long rotation);
/** The codec used by ImageCaptureHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `ImageCaptureHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(@NonNull BinaryMessenger binaryMessenger, @Nullable ImageCaptureHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.ImageCaptureHostApi.create", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Number targetRotationArg = (Number) args.get(1);
Number flashModeArg = (Number) args.get(2);
Number resolutionSelectorIdArg = (Number) args.get(3);
try {
api.create(
(identifierArg == null) ? null : identifierArg.longValue(),
(targetRotationArg == null) ? null : targetRotationArg.longValue(),
(flashModeArg == null) ? null : flashModeArg.longValue(),
(resolutionSelectorIdArg == null)
? null
: resolutionSelectorIdArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.ImageCaptureHostApi.setFlashMode", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Number flashModeArg = (Number) args.get(1);
try {
api.setFlashMode(
(identifierArg == null) ? null : identifierArg.longValue(),
(flashModeArg == null) ? null : flashModeArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.ImageCaptureHostApi.takePicture", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Result<String> resultCallback =
new Result<String>() {
public void success(String result) {
wrapped.add(0, result);
reply.reply(wrapped);
}
public void error(Throwable error) {
ArrayList<Object> wrappedError = wrapError(error);
reply.reply(wrappedError);
}
};
api.takePicture(
(identifierArg == null) ? null : identifierArg.longValue(), resultCallback);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.ImageCaptureHostApi.setTargetRotation",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Number rotationArg = (Number) args.get(1);
try {
api.setTargetRotation(
(identifierArg == null) ? null : identifierArg.longValue(),
(rotationArg == null) ? null : rotationArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
private static class ResolutionStrategyHostApiCodec extends StandardMessageCodec {
public static final ResolutionStrategyHostApiCodec INSTANCE =
new ResolutionStrategyHostApiCodec();
private ResolutionStrategyHostApiCodec() {}
@Override
protected Object readValueOfType(byte type, @NonNull ByteBuffer buffer) {
switch (type) {
case (byte) 128:
return ResolutionInfo.fromList((ArrayList<Object>) readValue(buffer));
default:
return super.readValueOfType(type, buffer);
}
}
@Override
protected void writeValue(@NonNull ByteArrayOutputStream stream, Object value) {
if (value instanceof ResolutionInfo) {
stream.write(128);
writeValue(stream, ((ResolutionInfo) value).toList());
} else {
super.writeValue(stream, value);
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface ResolutionStrategyHostApi {
void create(
@NonNull Long identifier, @Nullable ResolutionInfo boundSize, @Nullable Long fallbackRule);
/** The codec used by ResolutionStrategyHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return ResolutionStrategyHostApiCodec.INSTANCE;
}
/**
* Sets up an instance of `ResolutionStrategyHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(
@NonNull BinaryMessenger binaryMessenger, @Nullable ResolutionStrategyHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.ResolutionStrategyHostApi.create", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
ResolutionInfo boundSizeArg = (ResolutionInfo) args.get(1);
Number fallbackRuleArg = (Number) args.get(2);
try {
api.create(
(identifierArg == null) ? null : identifierArg.longValue(),
boundSizeArg,
(fallbackRuleArg == null) ? null : fallbackRuleArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface ResolutionSelectorHostApi {
void create(
@NonNull Long identifier,
@Nullable Long resolutionStrategyIdentifier,
@Nullable Long aspectRatioStrategyIdentifier);
/** The codec used by ResolutionSelectorHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `ResolutionSelectorHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(
@NonNull BinaryMessenger binaryMessenger, @Nullable ResolutionSelectorHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.ResolutionSelectorHostApi.create", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Number resolutionStrategyIdentifierArg = (Number) args.get(1);
Number aspectRatioStrategyIdentifierArg = (Number) args.get(2);
try {
api.create(
(identifierArg == null) ? null : identifierArg.longValue(),
(resolutionStrategyIdentifierArg == null)
? null
: resolutionStrategyIdentifierArg.longValue(),
(aspectRatioStrategyIdentifierArg == null)
? null
: aspectRatioStrategyIdentifierArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface AspectRatioStrategyHostApi {
void create(
@NonNull Long identifier, @NonNull Long preferredAspectRatio, @NonNull Long fallbackRule);
/** The codec used by AspectRatioStrategyHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `AspectRatioStrategyHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(
@NonNull BinaryMessenger binaryMessenger, @Nullable AspectRatioStrategyHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.AspectRatioStrategyHostApi.create",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Number preferredAspectRatioArg = (Number) args.get(1);
Number fallbackRuleArg = (Number) args.get(2);
try {
api.create(
(identifierArg == null) ? null : identifierArg.longValue(),
(preferredAspectRatioArg == null)
? null
: preferredAspectRatioArg.longValue(),
(fallbackRuleArg == null) ? null : fallbackRuleArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
private static class CameraStateFlutterApiCodec extends StandardMessageCodec {
public static final CameraStateFlutterApiCodec INSTANCE = new CameraStateFlutterApiCodec();
private CameraStateFlutterApiCodec() {}
@Override
protected Object readValueOfType(byte type, @NonNull ByteBuffer buffer) {
switch (type) {
case (byte) 128:
return CameraStateTypeData.fromList((ArrayList<Object>) readValue(buffer));
default:
return super.readValueOfType(type, buffer);
}
}
@Override
protected void writeValue(@NonNull ByteArrayOutputStream stream, Object value) {
if (value instanceof CameraStateTypeData) {
stream.write(128);
writeValue(stream, ((CameraStateTypeData) value).toList());
} else {
super.writeValue(stream, value);
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class CameraStateFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public CameraStateFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by CameraStateFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return CameraStateFlutterApiCodec.INSTANCE;
}
public void create(
@NonNull Long identifierArg,
@NonNull CameraStateTypeData typeArg,
@Nullable Long errorIdentifierArg,
@NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.CameraStateFlutterApi.create", getCodec());
channel.send(
new ArrayList<Object>(Arrays.asList(identifierArg, typeArg, errorIdentifierArg)),
channelReply -> callback.reply(null));
}
}
private static class ExposureStateFlutterApiCodec extends StandardMessageCodec {
public static final ExposureStateFlutterApiCodec INSTANCE = new ExposureStateFlutterApiCodec();
private ExposureStateFlutterApiCodec() {}
@Override
protected Object readValueOfType(byte type, @NonNull ByteBuffer buffer) {
switch (type) {
case (byte) 128:
return ExposureCompensationRange.fromList((ArrayList<Object>) readValue(buffer));
default:
return super.readValueOfType(type, buffer);
}
}
@Override
protected void writeValue(@NonNull ByteArrayOutputStream stream, Object value) {
if (value instanceof ExposureCompensationRange) {
stream.write(128);
writeValue(stream, ((ExposureCompensationRange) value).toList());
} else {
super.writeValue(stream, value);
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class ExposureStateFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public ExposureStateFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by ExposureStateFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return ExposureStateFlutterApiCodec.INSTANCE;
}
public void create(
@NonNull Long identifierArg,
@NonNull ExposureCompensationRange exposureCompensationRangeArg,
@NonNull Double exposureCompensationStepArg,
@NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.ExposureStateFlutterApi.create", getCodec());
channel.send(
new ArrayList<Object>(
Arrays.asList(
identifierArg, exposureCompensationRangeArg, exposureCompensationStepArg)),
channelReply -> callback.reply(null));
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class ZoomStateFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public ZoomStateFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by ZoomStateFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void create(
@NonNull Long identifierArg,
@NonNull Double minZoomRatioArg,
@NonNull Double maxZoomRatioArg,
@NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.ZoomStateFlutterApi.create", getCodec());
channel.send(
new ArrayList<Object>(Arrays.asList(identifierArg, minZoomRatioArg, maxZoomRatioArg)),
channelReply -> callback.reply(null));
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface ImageAnalysisHostApi {
void create(
@NonNull Long identifier,
@Nullable Long targetRotation,
@Nullable Long resolutionSelectorId);
void setAnalyzer(@NonNull Long identifier, @NonNull Long analyzerIdentifier);
void clearAnalyzer(@NonNull Long identifier);
void setTargetRotation(@NonNull Long identifier, @NonNull Long rotation);
/** The codec used by ImageAnalysisHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `ImageAnalysisHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(
@NonNull BinaryMessenger binaryMessenger, @Nullable ImageAnalysisHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.ImageAnalysisHostApi.create", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Number targetRotationArg = (Number) args.get(1);
Number resolutionSelectorIdArg = (Number) args.get(2);
try {
api.create(
(identifierArg == null) ? null : identifierArg.longValue(),
(targetRotationArg == null) ? null : targetRotationArg.longValue(),
(resolutionSelectorIdArg == null)
? null
: resolutionSelectorIdArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.ImageAnalysisHostApi.setAnalyzer", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Number analyzerIdentifierArg = (Number) args.get(1);
try {
api.setAnalyzer(
(identifierArg == null) ? null : identifierArg.longValue(),
(analyzerIdentifierArg == null) ? null : analyzerIdentifierArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.ImageAnalysisHostApi.clearAnalyzer",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
api.clearAnalyzer((identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.ImageAnalysisHostApi.setTargetRotation",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Number rotationArg = (Number) args.get(1);
try {
api.setTargetRotation(
(identifierArg == null) ? null : identifierArg.longValue(),
(rotationArg == null) ? null : rotationArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface AnalyzerHostApi {
void create(@NonNull Long identifier);
/** The codec used by AnalyzerHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `AnalyzerHostApi` to handle messages through the `binaryMessenger`.
*/
static void setup(@NonNull BinaryMessenger binaryMessenger, @Nullable AnalyzerHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.AnalyzerHostApi.create", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
api.create((identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface ObserverHostApi {
void create(@NonNull Long identifier);
/** The codec used by ObserverHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `ObserverHostApi` to handle messages through the `binaryMessenger`.
*/
static void setup(@NonNull BinaryMessenger binaryMessenger, @Nullable ObserverHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.ObserverHostApi.create", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
api.create((identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class ObserverFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public ObserverFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by ObserverFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void onChanged(
@NonNull Long identifierArg,
@NonNull Long valueIdentifierArg,
@NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.ObserverFlutterApi.onChanged", getCodec());
channel.send(
new ArrayList<Object>(Arrays.asList(identifierArg, valueIdentifierArg)),
channelReply -> callback.reply(null));
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class CameraStateErrorFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public CameraStateErrorFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by CameraStateErrorFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void create(
@NonNull Long identifierArg, @NonNull Long codeArg, @NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.CameraStateErrorFlutterApi.create", getCodec());
channel.send(
new ArrayList<Object>(Arrays.asList(identifierArg, codeArg)),
channelReply -> callback.reply(null));
}
}
private static class LiveDataHostApiCodec extends StandardMessageCodec {
public static final LiveDataHostApiCodec INSTANCE = new LiveDataHostApiCodec();
private LiveDataHostApiCodec() {}
@Override
protected Object readValueOfType(byte type, @NonNull ByteBuffer buffer) {
switch (type) {
case (byte) 128:
return LiveDataSupportedTypeData.fromList((ArrayList<Object>) readValue(buffer));
default:
return super.readValueOfType(type, buffer);
}
}
@Override
protected void writeValue(@NonNull ByteArrayOutputStream stream, Object value) {
if (value instanceof LiveDataSupportedTypeData) {
stream.write(128);
writeValue(stream, ((LiveDataSupportedTypeData) value).toList());
} else {
super.writeValue(stream, value);
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface LiveDataHostApi {
void observe(@NonNull Long identifier, @NonNull Long observerIdentifier);
void removeObservers(@NonNull Long identifier);
@Nullable
Long getValue(@NonNull Long identifier, @NonNull LiveDataSupportedTypeData type);
/** The codec used by LiveDataHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return LiveDataHostApiCodec.INSTANCE;
}
/**
* Sets up an instance of `LiveDataHostApi` to handle messages through the `binaryMessenger`.
*/
static void setup(@NonNull BinaryMessenger binaryMessenger, @Nullable LiveDataHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.LiveDataHostApi.observe", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Number observerIdentifierArg = (Number) args.get(1);
try {
api.observe(
(identifierArg == null) ? null : identifierArg.longValue(),
(observerIdentifierArg == null) ? null : observerIdentifierArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.LiveDataHostApi.removeObservers", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
api.removeObservers((identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.LiveDataHostApi.getValue", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
LiveDataSupportedTypeData typeArg = (LiveDataSupportedTypeData) args.get(1);
try {
Long output =
api.getValue(
(identifierArg == null) ? null : identifierArg.longValue(), typeArg);
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
private static class LiveDataFlutterApiCodec extends StandardMessageCodec {
public static final LiveDataFlutterApiCodec INSTANCE = new LiveDataFlutterApiCodec();
private LiveDataFlutterApiCodec() {}
@Override
protected Object readValueOfType(byte type, @NonNull ByteBuffer buffer) {
switch (type) {
case (byte) 128:
return LiveDataSupportedTypeData.fromList((ArrayList<Object>) readValue(buffer));
default:
return super.readValueOfType(type, buffer);
}
}
@Override
protected void writeValue(@NonNull ByteArrayOutputStream stream, Object value) {
if (value instanceof LiveDataSupportedTypeData) {
stream.write(128);
writeValue(stream, ((LiveDataSupportedTypeData) value).toList());
} else {
super.writeValue(stream, value);
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class LiveDataFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public LiveDataFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by LiveDataFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return LiveDataFlutterApiCodec.INSTANCE;
}
public void create(
@NonNull Long identifierArg,
@NonNull LiveDataSupportedTypeData typeArg,
@NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.LiveDataFlutterApi.create", getCodec());
channel.send(
new ArrayList<Object>(Arrays.asList(identifierArg, typeArg)),
channelReply -> callback.reply(null));
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class AnalyzerFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public AnalyzerFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by AnalyzerFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void create(@NonNull Long identifierArg, @NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.AnalyzerFlutterApi.create", getCodec());
channel.send(
new ArrayList<Object>(Collections.singletonList(identifierArg)),
channelReply -> callback.reply(null));
}
public void analyze(
@NonNull Long identifierArg,
@NonNull Long imageProxyIdentifierArg,
@NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.AnalyzerFlutterApi.analyze", getCodec());
channel.send(
new ArrayList<Object>(Arrays.asList(identifierArg, imageProxyIdentifierArg)),
channelReply -> callback.reply(null));
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface ImageProxyHostApi {
@NonNull
List<Long> getPlanes(@NonNull Long identifier);
void close(@NonNull Long identifier);
/** The codec used by ImageProxyHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `ImageProxyHostApi` to handle messages through the `binaryMessenger`.
*/
static void setup(@NonNull BinaryMessenger binaryMessenger, @Nullable ImageProxyHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.ImageProxyHostApi.getPlanes", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
List<Long> output =
api.getPlanes((identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.ImageProxyHostApi.close", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
api.close((identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class ImageProxyFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public ImageProxyFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by ImageProxyFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void create(
@NonNull Long identifierArg,
@NonNull Long formatArg,
@NonNull Long heightArg,
@NonNull Long widthArg,
@NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.ImageProxyFlutterApi.create", getCodec());
channel.send(
new ArrayList<Object>(Arrays.asList(identifierArg, formatArg, heightArg, widthArg)),
channelReply -> callback.reply(null));
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class PlaneProxyFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public PlaneProxyFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by PlaneProxyFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void create(
@NonNull Long identifierArg,
@NonNull byte[] bufferArg,
@NonNull Long pixelStrideArg,
@NonNull Long rowStrideArg,
@NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.PlaneProxyFlutterApi.create", getCodec());
channel.send(
new ArrayList<Object>(
Arrays.asList(identifierArg, bufferArg, pixelStrideArg, rowStrideArg)),
channelReply -> callback.reply(null));
}
}
private static class QualitySelectorHostApiCodec extends StandardMessageCodec {
public static final QualitySelectorHostApiCodec INSTANCE = new QualitySelectorHostApiCodec();
private QualitySelectorHostApiCodec() {}
@Override
protected Object readValueOfType(byte type, @NonNull ByteBuffer buffer) {
switch (type) {
case (byte) 128:
return ResolutionInfo.fromList((ArrayList<Object>) readValue(buffer));
case (byte) 129:
return VideoQualityData.fromList((ArrayList<Object>) readValue(buffer));
default:
return super.readValueOfType(type, buffer);
}
}
@Override
protected void writeValue(@NonNull ByteArrayOutputStream stream, Object value) {
if (value instanceof ResolutionInfo) {
stream.write(128);
writeValue(stream, ((ResolutionInfo) value).toList());
} else if (value instanceof VideoQualityData) {
stream.write(129);
writeValue(stream, ((VideoQualityData) value).toList());
} else {
super.writeValue(stream, value);
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface QualitySelectorHostApi {
void create(
@NonNull Long identifier,
@NonNull List<VideoQualityData> videoQualityDataList,
@Nullable Long fallbackStrategyId);
@NonNull
ResolutionInfo getResolution(@NonNull Long cameraInfoId, @NonNull VideoQuality quality);
/** The codec used by QualitySelectorHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return QualitySelectorHostApiCodec.INSTANCE;
}
/**
* Sets up an instance of `QualitySelectorHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(
@NonNull BinaryMessenger binaryMessenger, @Nullable QualitySelectorHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.QualitySelectorHostApi.create", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
List<VideoQualityData> videoQualityDataListArg =
(List<VideoQualityData>) args.get(1);
Number fallbackStrategyIdArg = (Number) args.get(2);
try {
api.create(
(identifierArg == null) ? null : identifierArg.longValue(),
videoQualityDataListArg,
(fallbackStrategyIdArg == null) ? null : fallbackStrategyIdArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.QualitySelectorHostApi.getResolution",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number cameraInfoIdArg = (Number) args.get(0);
VideoQuality qualityArg =
args.get(1) == null ? null : VideoQuality.values()[(int) args.get(1)];
try {
ResolutionInfo output =
api.getResolution(
(cameraInfoIdArg == null) ? null : cameraInfoIdArg.longValue(),
qualityArg);
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface FallbackStrategyHostApi {
void create(
@NonNull Long identifier,
@NonNull VideoQuality quality,
@NonNull VideoResolutionFallbackRule fallbackRule);
/** The codec used by FallbackStrategyHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `FallbackStrategyHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(
@NonNull BinaryMessenger binaryMessenger, @Nullable FallbackStrategyHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.FallbackStrategyHostApi.create", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
VideoQuality qualityArg =
args.get(1) == null ? null : VideoQuality.values()[(int) args.get(1)];
VideoResolutionFallbackRule fallbackRuleArg =
args.get(2) == null
? null
: VideoResolutionFallbackRule.values()[(int) args.get(2)];
try {
api.create(
(identifierArg == null) ? null : identifierArg.longValue(),
qualityArg,
fallbackRuleArg);
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface CameraControlHostApi {
void enableTorch(
@NonNull Long identifier, @NonNull Boolean torch, @NonNull Result<Void> result);
void setZoomRatio(
@NonNull Long identifier, @NonNull Double ratio, @NonNull Result<Void> result);
void startFocusAndMetering(
@NonNull Long identifier,
@NonNull Long focusMeteringActionId,
@NonNull Result<Long> result);
void cancelFocusAndMetering(@NonNull Long identifier, @NonNull Result<Void> result);
void setExposureCompensationIndex(
@NonNull Long identifier, @NonNull Long index, @NonNull Result<Long> result);
/** The codec used by CameraControlHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `CameraControlHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(
@NonNull BinaryMessenger binaryMessenger, @Nullable CameraControlHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.CameraControlHostApi.enableTorch", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Boolean torchArg = (Boolean) args.get(1);
Result<Void> resultCallback =
new Result<Void>() {
public void success(Void result) {
wrapped.add(0, null);
reply.reply(wrapped);
}
public void error(Throwable error) {
ArrayList<Object> wrappedError = wrapError(error);
reply.reply(wrappedError);
}
};
api.enableTorch(
(identifierArg == null) ? null : identifierArg.longValue(),
torchArg,
resultCallback);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.CameraControlHostApi.setZoomRatio",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Double ratioArg = (Double) args.get(1);
Result<Void> resultCallback =
new Result<Void>() {
public void success(Void result) {
wrapped.add(0, null);
reply.reply(wrapped);
}
public void error(Throwable error) {
ArrayList<Object> wrappedError = wrapError(error);
reply.reply(wrappedError);
}
};
api.setZoomRatio(
(identifierArg == null) ? null : identifierArg.longValue(),
ratioArg,
resultCallback);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.CameraControlHostApi.startFocusAndMetering",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Number focusMeteringActionIdArg = (Number) args.get(1);
Result<Long> resultCallback =
new Result<Long>() {
public void success(Long result) {
wrapped.add(0, result);
reply.reply(wrapped);
}
public void error(Throwable error) {
ArrayList<Object> wrappedError = wrapError(error);
reply.reply(wrappedError);
}
};
api.startFocusAndMetering(
(identifierArg == null) ? null : identifierArg.longValue(),
(focusMeteringActionIdArg == null)
? null
: focusMeteringActionIdArg.longValue(),
resultCallback);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.CameraControlHostApi.cancelFocusAndMetering",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Result<Void> resultCallback =
new Result<Void>() {
public void success(Void result) {
wrapped.add(0, null);
reply.reply(wrapped);
}
public void error(Throwable error) {
ArrayList<Object> wrappedError = wrapError(error);
reply.reply(wrappedError);
}
};
api.cancelFocusAndMetering(
(identifierArg == null) ? null : identifierArg.longValue(), resultCallback);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.CameraControlHostApi.setExposureCompensationIndex",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Number indexArg = (Number) args.get(1);
Result<Long> resultCallback =
new Result<Long>() {
public void success(Long result) {
wrapped.add(0, result);
reply.reply(wrapped);
}
public void error(Throwable error) {
ArrayList<Object> wrappedError = wrapError(error);
reply.reply(wrappedError);
}
};
api.setExposureCompensationIndex(
(identifierArg == null) ? null : identifierArg.longValue(),
(indexArg == null) ? null : indexArg.longValue(),
resultCallback);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class CameraControlFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public CameraControlFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by CameraControlFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void create(@NonNull Long identifierArg, @NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.CameraControlFlutterApi.create", getCodec());
channel.send(
new ArrayList<Object>(Collections.singletonList(identifierArg)),
channelReply -> callback.reply(null));
}
}
private static class FocusMeteringActionHostApiCodec extends StandardMessageCodec {
public static final FocusMeteringActionHostApiCodec INSTANCE =
new FocusMeteringActionHostApiCodec();
private FocusMeteringActionHostApiCodec() {}
@Override
protected Object readValueOfType(byte type, @NonNull ByteBuffer buffer) {
switch (type) {
case (byte) 128:
return MeteringPointInfo.fromList((ArrayList<Object>) readValue(buffer));
default:
return super.readValueOfType(type, buffer);
}
}
@Override
protected void writeValue(@NonNull ByteArrayOutputStream stream, Object value) {
if (value instanceof MeteringPointInfo) {
stream.write(128);
writeValue(stream, ((MeteringPointInfo) value).toList());
} else {
super.writeValue(stream, value);
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface FocusMeteringActionHostApi {
void create(
@NonNull Long identifier,
@NonNull List<MeteringPointInfo> meteringPointInfos,
@Nullable Boolean disableAutoCancel);
/** The codec used by FocusMeteringActionHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return FocusMeteringActionHostApiCodec.INSTANCE;
}
/**
* Sets up an instance of `FocusMeteringActionHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(
@NonNull BinaryMessenger binaryMessenger, @Nullable FocusMeteringActionHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.FocusMeteringActionHostApi.create",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
List<MeteringPointInfo> meteringPointInfosArg =
(List<MeteringPointInfo>) args.get(1);
Boolean disableAutoCancelArg = (Boolean) args.get(2);
try {
api.create(
(identifierArg == null) ? null : identifierArg.longValue(),
meteringPointInfosArg,
disableAutoCancelArg);
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface FocusMeteringResultHostApi {
@NonNull
Boolean isFocusSuccessful(@NonNull Long identifier);
/** The codec used by FocusMeteringResultHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `FocusMeteringResultHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(
@NonNull BinaryMessenger binaryMessenger, @Nullable FocusMeteringResultHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.FocusMeteringResultHostApi.isFocusSuccessful",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
try {
Boolean output =
api.isFocusSuccessful(
(identifierArg == null) ? null : identifierArg.longValue());
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated class from Pigeon that represents Flutter messages that can be called from Java. */
public static class FocusMeteringResultFlutterApi {
private final @NonNull BinaryMessenger binaryMessenger;
public FocusMeteringResultFlutterApi(@NonNull BinaryMessenger argBinaryMessenger) {
this.binaryMessenger = argBinaryMessenger;
}
/** Public interface for sending reply. */
@SuppressWarnings("UnknownNullness")
public interface Reply<T> {
void reply(T reply);
}
/** The codec used by FocusMeteringResultFlutterApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
public void create(@NonNull Long identifierArg, @NonNull Reply<Void> callback) {
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.FocusMeteringResultFlutterApi.create",
getCodec());
channel.send(
new ArrayList<Object>(Collections.singletonList(identifierArg)),
channelReply -> callback.reply(null));
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface MeteringPointHostApi {
void create(
@NonNull Long identifier,
@NonNull Double x,
@NonNull Double y,
@Nullable Double size,
@NonNull Long cameraInfoId);
@NonNull
Double getDefaultPointSize();
/** The codec used by MeteringPointHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `MeteringPointHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(
@NonNull BinaryMessenger binaryMessenger, @Nullable MeteringPointHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger, "dev.flutter.pigeon.MeteringPointHostApi.create", getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Double xArg = (Double) args.get(1);
Double yArg = (Double) args.get(2);
Double sizeArg = (Double) args.get(3);
Number cameraInfoIdArg = (Number) args.get(4);
try {
api.create(
(identifierArg == null) ? null : identifierArg.longValue(),
xArg,
yArg,
sizeArg,
(cameraInfoIdArg == null) ? null : cameraInfoIdArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.MeteringPointHostApi.getDefaultPointSize",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
try {
Double output = api.getDefaultPointSize();
wrapped.add(0, output);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
private static class CaptureRequestOptionsHostApiCodec extends StandardMessageCodec {
public static final CaptureRequestOptionsHostApiCodec INSTANCE =
new CaptureRequestOptionsHostApiCodec();
private CaptureRequestOptionsHostApiCodec() {}
@Override
protected Object readValueOfType(byte type, @NonNull ByteBuffer buffer) {
switch (type) {
case (byte) 128:
return CameraPermissionsErrorData.fromList((ArrayList<Object>) readValue(buffer));
case (byte) 129:
return CameraStateTypeData.fromList((ArrayList<Object>) readValue(buffer));
case (byte) 130:
return ExposureCompensationRange.fromList((ArrayList<Object>) readValue(buffer));
case (byte) 131:
return LiveDataSupportedTypeData.fromList((ArrayList<Object>) readValue(buffer));
case (byte) 132:
return MeteringPointInfo.fromList((ArrayList<Object>) readValue(buffer));
case (byte) 133:
return ResolutionInfo.fromList((ArrayList<Object>) readValue(buffer));
case (byte) 134:
return VideoQualityData.fromList((ArrayList<Object>) readValue(buffer));
default:
return super.readValueOfType(type, buffer);
}
}
@Override
protected void writeValue(@NonNull ByteArrayOutputStream stream, Object value) {
if (value instanceof CameraPermissionsErrorData) {
stream.write(128);
writeValue(stream, ((CameraPermissionsErrorData) value).toList());
} else if (value instanceof CameraStateTypeData) {
stream.write(129);
writeValue(stream, ((CameraStateTypeData) value).toList());
} else if (value instanceof ExposureCompensationRange) {
stream.write(130);
writeValue(stream, ((ExposureCompensationRange) value).toList());
} else if (value instanceof LiveDataSupportedTypeData) {
stream.write(131);
writeValue(stream, ((LiveDataSupportedTypeData) value).toList());
} else if (value instanceof MeteringPointInfo) {
stream.write(132);
writeValue(stream, ((MeteringPointInfo) value).toList());
} else if (value instanceof ResolutionInfo) {
stream.write(133);
writeValue(stream, ((ResolutionInfo) value).toList());
} else if (value instanceof VideoQualityData) {
stream.write(134);
writeValue(stream, ((VideoQualityData) value).toList());
} else {
super.writeValue(stream, value);
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface CaptureRequestOptionsHostApi {
void create(@NonNull Long identifier, @NonNull Map<Long, Object> options);
/** The codec used by CaptureRequestOptionsHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return CaptureRequestOptionsHostApiCodec.INSTANCE;
}
/**
* Sets up an instance of `CaptureRequestOptionsHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(
@NonNull BinaryMessenger binaryMessenger, @Nullable CaptureRequestOptionsHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.CaptureRequestOptionsHostApi.create",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Map<Long, Object> optionsArg = (Map<Long, Object>) args.get(1);
try {
api.create(
(identifierArg == null) ? null : identifierArg.longValue(), optionsArg);
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
/** Generated interface from Pigeon that represents a handler of messages from Flutter. */
public interface Camera2CameraControlHostApi {
void create(@NonNull Long identifier, @NonNull Long cameraControlIdentifier);
void addCaptureRequestOptions(
@NonNull Long identifier,
@NonNull Long captureRequestOptionsIdentifier,
@NonNull Result<Void> result);
/** The codec used by Camera2CameraControlHostApi. */
static @NonNull MessageCodec<Object> getCodec() {
return new StandardMessageCodec();
}
/**
* Sets up an instance of `Camera2CameraControlHostApi` to handle messages through the
* `binaryMessenger`.
*/
static void setup(
@NonNull BinaryMessenger binaryMessenger, @Nullable Camera2CameraControlHostApi api) {
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.Camera2CameraControlHostApi.create",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Number cameraControlIdentifierArg = (Number) args.get(1);
try {
api.create(
(identifierArg == null) ? null : identifierArg.longValue(),
(cameraControlIdentifierArg == null)
? null
: cameraControlIdentifierArg.longValue());
wrapped.add(0, null);
} catch (Throwable exception) {
ArrayList<Object> wrappedError = wrapError(exception);
wrapped = wrappedError;
}
reply.reply(wrapped);
});
} else {
channel.setMessageHandler(null);
}
}
{
BasicMessageChannel<Object> channel =
new BasicMessageChannel<>(
binaryMessenger,
"dev.flutter.pigeon.Camera2CameraControlHostApi.addCaptureRequestOptions",
getCodec());
if (api != null) {
channel.setMessageHandler(
(message, reply) -> {
ArrayList<Object> wrapped = new ArrayList<Object>();
ArrayList<Object> args = (ArrayList<Object>) message;
Number identifierArg = (Number) args.get(0);
Number captureRequestOptionsIdentifierArg = (Number) args.get(1);
Result<Void> resultCallback =
new Result<Void>() {
public void success(Void result) {
wrapped.add(0, null);
reply.reply(wrapped);
}
public void error(Throwable error) {
ArrayList<Object> wrappedError = wrapError(error);
reply.reply(wrappedError);
}
};
api.addCaptureRequestOptions(
(identifierArg == null) ? null : identifierArg.longValue(),
(captureRequestOptionsIdentifierArg == null)
? null
: captureRequestOptionsIdentifierArg.longValue(),
resultCallback);
});
} else {
channel.setMessageHandler(null);
}
}
}
}
}
| packages/packages/camera/camera_android_camerax/android/src/main/java/io/flutter/plugins/camerax/GeneratedCameraXLibrary.java/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/android/src/main/java/io/flutter/plugins/camerax/GeneratedCameraXLibrary.java",
"repo_id": "packages",
"token_count": 70100
} | 900 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.camerax;
import static org.junit.Assert.assertEquals;
import static org.mockito.Mockito.when;
import androidx.camera.core.resolutionselector.AspectRatioStrategy;
import org.junit.After;
import org.junit.Before;
import org.junit.Rule;
import org.junit.Test;
import org.mockito.Mock;
import org.mockito.junit.MockitoJUnit;
import org.mockito.junit.MockitoRule;
public class AspectRatioStrategyTest {
@Rule public MockitoRule mockitoRule = MockitoJUnit.rule();
@Mock public AspectRatioStrategy mockAspectRatioStrategy;
@Mock public AspectRatioStrategyHostApiImpl.AspectRatioStrategyProxy mockProxy;
InstanceManager instanceManager;
@Before
public void setUp() {
instanceManager = InstanceManager.create(identifier -> {});
}
@After
public void tearDown() {
instanceManager.stopFinalizationListener();
}
@Test
public void hostApiCreate_createsExpectedAspectRatioStrategyInstance() {
final Long preferredAspectRatio = 0L;
final Long fallbackRule = 1L;
when(mockProxy.create(preferredAspectRatio, fallbackRule)).thenReturn(mockAspectRatioStrategy);
final AspectRatioStrategyHostApiImpl hostApi =
new AspectRatioStrategyHostApiImpl(instanceManager, mockProxy);
final long instanceIdentifier = 0;
hostApi.create(instanceIdentifier, preferredAspectRatio, fallbackRule);
assertEquals(instanceManager.getInstance(instanceIdentifier), mockAspectRatioStrategy);
}
}
| packages/packages/camera/camera_android_camerax/android/src/test/java/io/flutter/plugins/camerax/AspectRatioStrategyTest.java/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/android/src/test/java/io/flutter/plugins/camerax/AspectRatioStrategyTest.java",
"repo_id": "packages",
"token_count": 534
} | 901 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.camerax;
import static org.junit.Assert.assertTrue;
import static org.mockito.ArgumentMatchers.any;
import static org.mockito.ArgumentMatchers.eq;
import static org.mockito.Mockito.verify;
import static org.mockito.Mockito.when;
import androidx.camera.core.FocusMeteringResult;
import io.flutter.plugin.common.BinaryMessenger;
import io.flutter.plugins.camerax.GeneratedCameraXLibrary.FocusMeteringResultFlutterApi;
import java.util.Objects;
import org.junit.After;
import org.junit.Before;
import org.junit.Rule;
import org.junit.Test;
import org.mockito.Mock;
import org.mockito.junit.MockitoJUnit;
import org.mockito.junit.MockitoRule;
public class FocusMeteringResultTest {
@Rule public MockitoRule mockitoRule = MockitoJUnit.rule();
@Mock public BinaryMessenger mockBinaryMessenger;
@Mock public FocusMeteringResult focusMeteringResult;
@Mock public FocusMeteringResultFlutterApi mockFlutterApi;
InstanceManager testInstanceManager;
@Before
public void setUp() {
testInstanceManager = InstanceManager.create(identifier -> {});
}
@After
public void tearDown() {
testInstanceManager.stopFinalizationListener();
}
@Test
public void isFocusSuccessful_returnsExpectedResult() {
final FocusMeteringResultHostApiImpl focusMeteringResultHostApiImpl =
new FocusMeteringResultHostApiImpl(testInstanceManager);
final Long focusMeteringResultIdentifier = 98L;
final boolean result = true;
testInstanceManager.addDartCreatedInstance(focusMeteringResult, focusMeteringResultIdentifier);
when(focusMeteringResult.isFocusSuccessful()).thenReturn(result);
assertTrue(focusMeteringResultHostApiImpl.isFocusSuccessful(focusMeteringResultIdentifier));
verify(focusMeteringResult).isFocusSuccessful();
}
@Test
public void flutterApiCreate_makesCallToCreateInstanceOnDartSide() {
final FocusMeteringResultFlutterApiImpl flutterApi =
new FocusMeteringResultFlutterApiImpl(mockBinaryMessenger, testInstanceManager);
flutterApi.setApi(mockFlutterApi);
flutterApi.create(focusMeteringResult, reply -> {});
final long focusMeteringResultIdentifier =
Objects.requireNonNull(
testInstanceManager.getIdentifierForStrongReference(focusMeteringResult));
verify(mockFlutterApi).create(eq(focusMeteringResultIdentifier), any());
}
}
| packages/packages/camera/camera_android_camerax/android/src/test/java/io/flutter/plugins/camerax/FocusMeteringResultTest.java/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/android/src/test/java/io/flutter/plugins/camerax/FocusMeteringResultTest.java",
"repo_id": "packages",
"token_count": 816
} | 902 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.camerax;
import static org.mockito.ArgumentMatchers.any;
import static org.mockito.ArgumentMatchers.eq;
import static org.mockito.Mockito.spy;
import static org.mockito.Mockito.verify;
import androidx.camera.video.Recording;
import io.flutter.plugin.common.BinaryMessenger;
import java.util.Objects;
import org.junit.After;
import org.junit.Before;
import org.junit.Rule;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.mockito.Mock;
import org.mockito.junit.MockitoJUnit;
import org.mockito.junit.MockitoRule;
import org.robolectric.RobolectricTestRunner;
@RunWith(RobolectricTestRunner.class)
public class RecordingTest {
@Rule public MockitoRule mockitoRule = MockitoJUnit.rule();
@Mock public BinaryMessenger mockBinaryMessenger;
@Mock public Recording mockRecording;
InstanceManager testInstanceManager;
@Before
public void setUp() {
testInstanceManager = spy(InstanceManager.create(identifier -> {}));
}
@After
public void tearDown() {
testInstanceManager.stopFinalizationListener();
}
@Test
public void close_getsRecordingFromInstanceManagerAndCloses() {
final RecordingHostApiImpl recordingHostApi =
new RecordingHostApiImpl(mockBinaryMessenger, testInstanceManager);
final Long recordingId = 5L;
testInstanceManager.addDartCreatedInstance(mockRecording, recordingId);
recordingHostApi.close(recordingId);
verify(mockRecording).close();
testInstanceManager.remove(recordingId);
}
@Test
public void stop_getsRecordingFromInstanceManagerAndStops() {
final RecordingHostApiImpl recordingHostApi =
new RecordingHostApiImpl(mockBinaryMessenger, testInstanceManager);
final Long recordingId = 5L;
testInstanceManager.addDartCreatedInstance(mockRecording, recordingId);
recordingHostApi.stop(recordingId);
verify(mockRecording).stop();
testInstanceManager.remove(recordingId);
}
@Test
public void resume_getsRecordingFromInstanceManagerAndResumes() {
final RecordingHostApiImpl recordingHostApi =
new RecordingHostApiImpl(mockBinaryMessenger, testInstanceManager);
final Long recordingId = 5L;
testInstanceManager.addDartCreatedInstance(mockRecording, recordingId);
recordingHostApi.resume(recordingId);
verify(mockRecording).resume();
testInstanceManager.remove(recordingId);
}
@Test
public void pause_getsRecordingFromInstanceManagerAndPauses() {
final RecordingHostApiImpl recordingHostApi =
new RecordingHostApiImpl(mockBinaryMessenger, testInstanceManager);
final Long recordingId = 5L;
testInstanceManager.addDartCreatedInstance(mockRecording, recordingId);
recordingHostApi.pause(recordingId);
verify(mockRecording).pause();
testInstanceManager.remove(recordingId);
}
@Test
public void flutterApiCreateTest() {
final RecordingFlutterApiImpl spyRecordingFlutterApi =
spy(new RecordingFlutterApiImpl(mockBinaryMessenger, testInstanceManager));
spyRecordingFlutterApi.create(mockRecording, reply -> {});
final long identifier =
Objects.requireNonNull(testInstanceManager.getIdentifierForStrongReference(mockRecording));
verify(spyRecordingFlutterApi).create(eq(identifier), any());
}
}
| packages/packages/camera/camera_android_camerax/android/src/test/java/io/flutter/plugins/camerax/RecordingTest.java/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/android/src/test/java/io/flutter/plugins/camerax/RecordingTest.java",
"repo_id": "packages",
"token_count": 1124
} | 903 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart';
import 'package:meta/meta.dart';
import 'camera_state.dart';
import 'camerax_library.g.dart';
import 'instance_manager.dart';
import 'java_object.dart';
/// The error that a camera has encountered.
///
/// See https://developer.android.com/reference/androidx/camera/core/CameraState.StateError.
@immutable
class CameraStateError extends JavaObject {
/// Constructs a [CameraStateError] that is not automatically attached to a native object.
CameraStateError.detached(
{super.binaryMessenger, super.instanceManager, required this.code})
: super.detached();
/// The code of this error.
///
/// Will map to one of the [CameraState] error codes that map to the CameraX
/// CameraState codes:
/// https://developer.android.com/reference/androidx/camera/core/CameraState#constants_1.
final int code;
/// Gets a description of this error corresponding to its [code].
///
/// This is not directly provided by the CameraX library, but is determined
/// based on the description of the [code].
///
/// Provided for developers to use for error handling.
String getDescription() {
String description = '';
switch (code) {
case CameraState.errorCameraInUse:
description =
'The camera was already in use, possibly by a higher-priority camera client.';
case CameraState.errorMaxCamerasInUse:
description =
'The limit number of open cameras has been reached, and more cameras cannot be opened until other instances are closed.';
case CameraState.errorOtherRecoverableError:
description =
'The camera device has encountered a recoverable error. CameraX will attempt to recover from the error.';
case CameraState.errorStreamConfig:
description = 'Configuring the camera has failed.';
case CameraState.errorCameraDisabled:
description =
'The camera device could not be opened due to a device policy. Thia may be caused by a client from a background process attempting to open the camera.';
case CameraState.errorCameraFatalError:
description =
'The camera was closed due to a fatal error. This may require the Android device be shut down and restarted to restore camera function or may indicate a persistent camera hardware problem.';
case CameraState.errorDoNotDisturbModeEnabled:
description =
'The camera could not be opened because "Do Not Disturb" mode is enabled. Please disable this mode, and try opening the camera again.';
default:
description =
'There was an unspecified issue with the current camera state.';
break;
}
return '$code : $description';
}
}
/// Flutter API implementation for [CameraStateError].
///
/// This class may handle instantiating and adding Dart instances that are
/// attached to a native instance or receiving callback methods from an
/// overridden native class.
@protected
class CameraStateErrorFlutterApiImpl implements CameraStateErrorFlutterApi {
/// Constructs a [CameraStateErrorFlutterApiImpl].
///
/// If [binaryMessenger] is null, the default [BinaryMessenger] will be used,
/// which routes to the host platform.
///
/// An [instanceManager] is typically passed when a copy of an instance
/// contained by an [InstanceManager] is being created. If left null, it
/// will default to the global instance defined in [JavaObject]. If left null, it
/// will default to the global instance defined in [JavaObject].
CameraStateErrorFlutterApiImpl({
BinaryMessenger? binaryMessenger,
InstanceManager? instanceManager,
}) : _binaryMessenger = binaryMessenger,
_instanceManager = instanceManager ?? JavaObject.globalInstanceManager;
/// Receives binary data across the Flutter platform barrier.
final BinaryMessenger? _binaryMessenger;
/// Maintains instances stored to communicate with native language objects.
final InstanceManager _instanceManager;
@override
void create(
int identifier,
int code,
) {
_instanceManager.addHostCreatedInstance(
CameraStateError.detached(
code: code,
binaryMessenger: _binaryMessenger,
instanceManager: _instanceManager,
),
identifier,
onCopy: (CameraStateError original) => CameraStateError.detached(
code: original.code,
binaryMessenger: _binaryMessenger,
instanceManager: _instanceManager,
),
);
}
}
| packages/packages/camera/camera_android_camerax/lib/src/camera_state_error.dart/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/lib/src/camera_state_error.dart",
"repo_id": "packages",
"token_count": 1414
} | 904 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/services.dart';
import 'package:meta/meta.dart';
import 'android_camera_camerax_flutter_api_impls.dart';
import 'camerax_library.g.dart';
import 'instance_manager.dart';
import 'java_object.dart';
import 'live_data.dart';
/// Callback that can receive from [LiveData].
///
/// See https://developer.android.com/reference/androidx/lifecycle/Observer.
@immutable
class Observer<T> extends JavaObject {
/// Constructor for [Observer].
Observer(
{super.binaryMessenger,
super.instanceManager,
required void Function(Object value) onChanged})
: _api = _ObserverHostApiImpl(
binaryMessenger: binaryMessenger, instanceManager: instanceManager),
super.detached() {
AndroidCameraXCameraFlutterApis.instance.ensureSetUp();
this.onChanged = (Object value) {
if (value is! T) {
throw ArgumentError(
'The type of value observed does not match the type of Observer constructed.');
}
onChanged(value);
};
_api.createFromInstance(this);
}
/// Constructs a [Observer] that is not automatically attached to a native object.
Observer.detached(
{super.binaryMessenger,
super.instanceManager,
required void Function(Object value) onChanged})
: _api = _ObserverHostApiImpl(
binaryMessenger: binaryMessenger, instanceManager: instanceManager),
super.detached() {
this.onChanged = (Object value) {
assert(value is T);
onChanged(value);
};
}
final _ObserverHostApiImpl _api;
/// Callback used when the observed data is changed to a new value.
///
/// The callback parameter cannot take type [T] directly due to the issue
/// described in https://github.com/dart-lang/sdk/issues/51461.
late final void Function(Object value) onChanged;
}
class _ObserverHostApiImpl extends ObserverHostApi {
/// Constructs an [_ObserverHostApiImpl].
///
/// If [binaryMessenger] is null, the default [BinaryMessenger] will be used,
/// which routes to the host platform.
///
/// An [instanceManager] is typically passed when a copy of an instance
/// contained by an [InstanceManager] is being created. If left null, it
/// will default to the global instance defined in [JavaObject].
_ObserverHostApiImpl({
this.binaryMessenger,
InstanceManager? instanceManager,
}) : instanceManager = instanceManager ?? JavaObject.globalInstanceManager,
super(binaryMessenger: binaryMessenger);
final BinaryMessenger? binaryMessenger;
final InstanceManager instanceManager;
/// Adds specified [Observer] instance to instance manager and makes call
/// to native side to create the instance.
Future<void> createFromInstance<T>(
Observer<T> instance,
) {
return create(
instanceManager.addDartCreatedInstance(
instance,
onCopy: (Observer<T> original) => Observer<T>.detached(
onChanged: original.onChanged,
binaryMessenger: binaryMessenger,
instanceManager: instanceManager,
),
),
);
}
}
/// Flutter API implementation for [Observer].
///
/// This class may handle instantiating and adding Dart instances that are
/// attached to a native instance or receiving callback methods from an
/// overridden native class.
@protected
class ObserverFlutterApiImpl implements ObserverFlutterApi {
/// Constructs an [ObserverFlutterApiImpl].
///
/// An [instanceManager] is typically passed when a copy of an instance
/// contained by an [InstanceManager] is being created. If left null, it
/// will default to the global instance defined in [JavaObject].
ObserverFlutterApiImpl({
InstanceManager? instanceManager,
}) : _instanceManager = instanceManager ?? JavaObject.globalInstanceManager;
/// Maintains instances stored to communicate with native language objects.
final InstanceManager _instanceManager;
@override
void onChanged(
int identifier,
int valueIdentifier,
) {
final Observer<dynamic> instance =
_instanceManager.getInstanceWithWeakReference(identifier)!;
// This call is safe because the onChanged callback will check the type
// of the instance to ensure it is expected before proceeding.
// ignore: avoid_dynamic_calls, void_checks
instance.onChanged(
_instanceManager.getInstanceWithWeakReference<Object>(valueIdentifier)!,
);
}
}
| packages/packages/camera/camera_android_camerax/lib/src/observer.dart/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/lib/src/observer.dart",
"repo_id": "packages",
"token_count": 1440
} | 905 |
name: camera_android_camerax
description: Android implementation of the camera plugin using the CameraX library.
repository: https://github.com/flutter/packages/tree/main/packages/camera/camera_android_camerax
issue_tracker: https://github.com/flutter/flutter/issues?q=is%3Aissue+is%3Aopen+label%3A%22p%3A+camera%22
version: 0.6.0+1
environment:
sdk: ^3.1.0
flutter: ">=3.13.0"
flutter:
plugin:
implements: camera
platforms:
android:
package: io.flutter.plugins.camerax
pluginClass: CameraAndroidCameraxPlugin
dartPluginClass: AndroidCameraCameraX
dependencies:
async: ^2.5.0
camera_platform_interface: ^2.3.2
flutter:
sdk: flutter
integration_test:
sdk: flutter
meta: ^1.7.0
stream_transform: ^2.1.0
dev_dependencies:
build_runner: ^2.2.0
flutter_test:
sdk: flutter
mockito: 5.4.4
pigeon: ^9.1.0
topics:
- camera
| packages/packages/camera/camera_android_camerax/pubspec.yaml/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/pubspec.yaml",
"repo_id": "packages",
"token_count": 370
} | 906 |
// Mocks generated by Mockito 5.4.4 from annotations
// in camera_android_camerax/test/focus_metering_result_test.dart.
// Do not manually edit this file.
// ignore_for_file: no_leading_underscores_for_library_prefixes
import 'package:camera_android_camerax/src/camera_info.dart' as _i2;
import 'package:camera_android_camerax/src/metering_point.dart' as _i3;
import 'package:mockito/mockito.dart' as _i1;
import 'test_camerax_library.g.dart' as _i4;
// ignore_for_file: type=lint
// ignore_for_file: avoid_redundant_argument_values
// ignore_for_file: avoid_setters_without_getters
// ignore_for_file: comment_references
// ignore_for_file: deprecated_member_use
// ignore_for_file: deprecated_member_use_from_same_package
// ignore_for_file: implementation_imports
// ignore_for_file: invalid_use_of_visible_for_testing_member
// ignore_for_file: prefer_const_constructors
// ignore_for_file: unnecessary_parenthesis
// ignore_for_file: camel_case_types
// ignore_for_file: subtype_of_sealed_class
class _FakeCameraInfo_0 extends _i1.SmartFake implements _i2.CameraInfo {
_FakeCameraInfo_0(
Object parent,
Invocation parentInvocation,
) : super(
parent,
parentInvocation,
);
}
/// A class which mocks [MeteringPoint].
///
/// See the documentation for Mockito's code generation for more information.
// ignore: must_be_immutable
class MockMeteringPoint extends _i1.Mock implements _i3.MeteringPoint {
MockMeteringPoint() {
_i1.throwOnMissingStub(this);
}
@override
double get x => (super.noSuchMethod(
Invocation.getter(#x),
returnValue: 0.0,
) as double);
@override
double get y => (super.noSuchMethod(
Invocation.getter(#y),
returnValue: 0.0,
) as double);
@override
_i2.CameraInfo get cameraInfo => (super.noSuchMethod(
Invocation.getter(#cameraInfo),
returnValue: _FakeCameraInfo_0(
this,
Invocation.getter(#cameraInfo),
),
) as _i2.CameraInfo);
}
/// A class which mocks [TestFocusMeteringResultHostApi].
///
/// See the documentation for Mockito's code generation for more information.
class MockTestFocusMeteringResultHostApi extends _i1.Mock
implements _i4.TestFocusMeteringResultHostApi {
MockTestFocusMeteringResultHostApi() {
_i1.throwOnMissingStub(this);
}
@override
bool isFocusSuccessful(int? identifier) => (super.noSuchMethod(
Invocation.method(
#isFocusSuccessful,
[identifier],
),
returnValue: false,
) as bool);
}
/// A class which mocks [TestInstanceManagerHostApi].
///
/// See the documentation for Mockito's code generation for more information.
class MockTestInstanceManagerHostApi extends _i1.Mock
implements _i4.TestInstanceManagerHostApi {
MockTestInstanceManagerHostApi() {
_i1.throwOnMissingStub(this);
}
@override
void clear() => super.noSuchMethod(
Invocation.method(
#clear,
[],
),
returnValueForMissingStub: null,
);
}
| packages/packages/camera/camera_android_camerax/test/focus_metering_result_test.mocks.dart/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/test/focus_metering_result_test.mocks.dart",
"repo_id": "packages",
"token_count": 1160
} | 907 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:typed_data';
import 'package:camera_android_camerax/src/instance_manager.dart';
import 'package:camera_android_camerax/src/plane_proxy.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:mockito/annotations.dart';
import 'plane_proxy_test.mocks.dart';
import 'test_camerax_library.g.dart';
@GenerateMocks(<Type>[TestInstanceManagerHostApi])
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
// Mocks the call to clear the native InstanceManager.
TestInstanceManagerHostApi.setup(MockTestInstanceManagerHostApi());
group('PlaneProxy', () {
test('FlutterAPI create', () {
final InstanceManager instanceManager = InstanceManager(
onWeakReferenceRemoved: (_) {},
);
final PlaneProxyFlutterApiImpl api = PlaneProxyFlutterApiImpl(
instanceManager: instanceManager,
);
const int instanceIdentifier = 0;
final Uint8List buffer = Uint8List(1);
const int pixelStride = 3;
const int rowStride = 6;
api.create(instanceIdentifier, buffer, pixelStride, rowStride);
final PlaneProxy planeProxy =
instanceManager.getInstanceWithWeakReference(instanceIdentifier)!;
expect(planeProxy.buffer, equals(buffer));
expect(planeProxy.pixelStride, equals(pixelStride));
expect(planeProxy.rowStride, equals(rowStride));
});
});
}
| packages/packages/camera/camera_android_camerax/test/plane_proxy_test.dart/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/test/plane_proxy_test.dart",
"repo_id": "packages",
"token_count": 536
} | 908 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:camera_android_camerax/src/camerax_library.g.dart'
show CameraPermissionsErrorData;
import 'package:camera_android_camerax/src/system_services.dart';
import 'package:camera_platform_interface/camera_platform_interface.dart'
show CameraException;
import 'package:flutter_test/flutter_test.dart';
import 'package:mockito/annotations.dart';
import 'package:mockito/mockito.dart';
import 'system_services_test.mocks.dart';
import 'test_camerax_library.g.dart';
@GenerateMocks(<Type>[TestInstanceManagerHostApi, TestSystemServicesHostApi])
void main() {
TestWidgetsFlutterBinding.ensureInitialized();
// Mocks the call to clear the native InstanceManager.
TestInstanceManagerHostApi.setup(MockTestInstanceManagerHostApi());
group('SystemServices', () {
tearDown(() => TestProcessCameraProviderHostApi.setup(null));
test(
'requestCameraPermissionsFromInstance completes normally without errors test',
() async {
final MockTestSystemServicesHostApi mockApi =
MockTestSystemServicesHostApi();
TestSystemServicesHostApi.setup(mockApi);
when(mockApi.requestCameraPermissions(true))
.thenAnswer((_) async => null);
await SystemServices.requestCameraPermissions(true);
verify(mockApi.requestCameraPermissions(true));
});
test(
'requestCameraPermissionsFromInstance throws CameraException if there was a request error',
() {
final MockTestSystemServicesHostApi mockApi =
MockTestSystemServicesHostApi();
TestSystemServicesHostApi.setup(mockApi);
final CameraPermissionsErrorData error = CameraPermissionsErrorData(
errorCode: 'Test error code',
description: 'Test error description',
);
when(mockApi.requestCameraPermissions(true))
.thenAnswer((_) async => error);
expect(
() async => SystemServices.requestCameraPermissions(true),
throwsA(isA<CameraException>()
.having((CameraException e) => e.code, 'code', 'Test error code')
.having((CameraException e) => e.description, 'description',
'Test error description')));
verify(mockApi.requestCameraPermissions(true));
});
test('onCameraError adds new error to stream', () {
const String testErrorDescription = 'Test error description!';
SystemServices.cameraErrorStreamController.stream
.listen((String errorDescription) {
expect(errorDescription, equals(testErrorDescription));
});
SystemServicesFlutterApiImpl().onCameraError(testErrorDescription);
});
test('getTempFilePath completes normally', () async {
final MockTestSystemServicesHostApi mockApi =
MockTestSystemServicesHostApi();
TestSystemServicesHostApi.setup(mockApi);
const String testPath = '/test/path/';
const String testPrefix = 'MOV';
const String testSuffix = '.mp4';
when(mockApi.getTempFilePath(testPrefix, testSuffix))
.thenReturn(testPath + testPrefix + testSuffix);
expect(await SystemServices.getTempFilePath(testPrefix, testSuffix),
testPath + testPrefix + testSuffix);
verify(mockApi.getTempFilePath(testPrefix, testSuffix));
});
});
}
| packages/packages/camera/camera_android_camerax/test/system_services_test.dart/0 | {
"file_path": "packages/packages/camera/camera_android_camerax/test/system_services_test.dart",
"repo_id": "packages",
"token_count": 1238
} | 909 |
# cross_file
An abstraction to allow working with files across multiple platforms.
## Usage
Import `package:cross_file/cross_file.dart`, instantiate a `XFile`
using a path or byte array and use its methods and properties to
access the file and its metadata.
Example:
<?code-excerpt "example/lib/readme_excerpts.dart (Instantiate)"?>
```dart
final XFile file = XFile('assets/hello.txt');
print('File information:');
print('- Path: ${file.path}');
print('- Name: ${file.name}');
print('- MIME type: ${file.mimeType}');
final String fileContent = await file.readAsString();
print('Content of the file: $fileContent');
```
You will find links to the API docs on the [pub page](https://pub.dev/packages/cross_file).
## Web Limitations
`XFile` on the web platform is backed by [Blob](https://api.dart.dev/be/180361/dart-html/Blob-class.html)
objects and their URLs.
It seems that Safari hangs when reading Blobs larger than 4GB (your app will stop
without returning any data, or throwing an exception).
This package will attempt to throw an `Exception` before a large file is accessed
from Safari (if its size is known beforehand), so that case can be handled
programmatically.
### Browser compatibility
[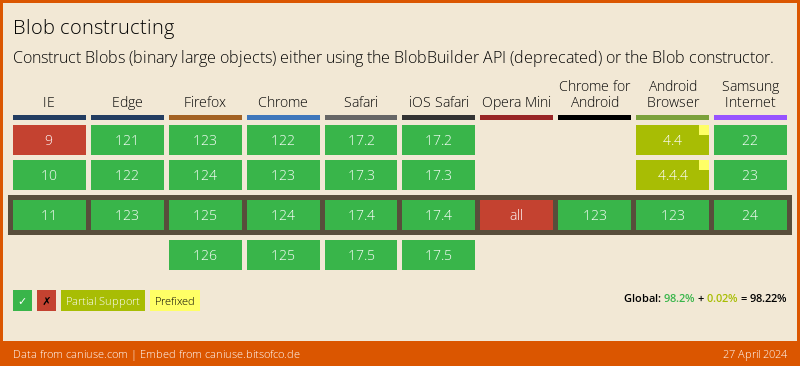](https://caniuse.com/blobbuilder)
[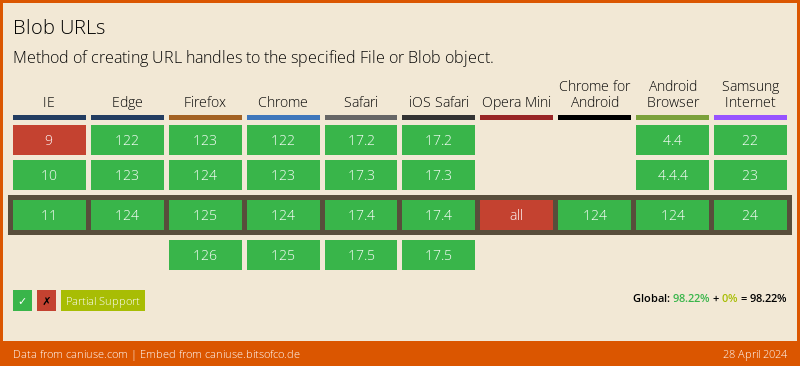](https://caniuse.com/bloburls)
## Testing
This package supports both web and native platforms. Unit tests need to be split
in two separate suites (because native code cannot use `dart:html`, and web code
cannot use `dart:io`).
When adding new features, it is likely that tests need to be added for both the
native and web platforms.
### Native tests
Tests for native platforms are located in the `x_file_io_test.dart`. Tests can
be run with `dart test`.
### Web tests
Tests for the web platform live in the `x_file_html_test.dart`. They can be run
with `dart test -p chrome`.
| packages/packages/cross_file/README.md/0 | {
"file_path": "packages/packages/cross_file/README.md",
"repo_id": "packages",
"token_count": 611
} | 910 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
@TestOn('vm') // Uses dart:io
library;
import 'dart:convert';
import 'dart:io';
import 'dart:typed_data';
import 'package:cross_file/cross_file.dart';
import 'package:test/test.dart';
final String pathPrefix =
Directory.current.path.endsWith('test') ? './assets/' : './test/assets/';
final String path = '${pathPrefix}hello.txt';
const String expectedStringContents = 'Hello, world!';
final Uint8List bytes = Uint8List.fromList(utf8.encode(expectedStringContents));
final File textFile = File(path);
final String textFilePath = textFile.path;
void main() {
group('Create with a path', () {
test('Can be read as a string', () async {
final XFile file = XFile(textFilePath);
expect(await file.readAsString(), equals(expectedStringContents));
});
test('Can be read as bytes', () async {
final XFile file = XFile(textFilePath);
expect(await file.readAsBytes(), equals(bytes));
});
test('Can be read as a stream', () async {
final XFile file = XFile(textFilePath);
expect(await file.openRead().first, equals(bytes));
});
test('Stream can be sliced', () async {
final XFile file = XFile(textFilePath);
expect(await file.openRead(2, 5).first, equals(bytes.sublist(2, 5)));
});
test('saveTo(..) creates file', () async {
final XFile file = XFile(textFilePath);
final Directory tempDir = Directory.systemTemp.createTempSync();
final File targetFile = File('${tempDir.path}/newFilePath.txt');
if (targetFile.existsSync()) {
await targetFile.delete();
}
await file.saveTo(targetFile.path);
expect(targetFile.existsSync(), isTrue);
expect(targetFile.readAsStringSync(), 'Hello, world!');
await tempDir.delete(recursive: true);
});
test('saveTo(..) does not load the file into memory', () async {
final TestXFile file = TestXFile(textFilePath);
final Directory tempDir = Directory.systemTemp.createTempSync();
final File targetFile = File('${tempDir.path}/newFilePath.txt');
if (targetFile.existsSync()) {
await targetFile.delete();
}
await file.saveTo(targetFile.path);
expect(file.hasBeenRead, isFalse);
await tempDir.delete(recursive: true);
});
test('nullability is correct', () async {
expect(_ensureNonnullPathArgument('a/path'), isNotNull);
});
});
group('Create with data', () {
final XFile file = XFile.fromData(bytes);
test('Can be read as a string', () async {
expect(await file.readAsString(), equals(expectedStringContents));
});
test('Can be read as bytes', () async {
expect(await file.readAsBytes(), equals(bytes));
});
test('Can be read as a stream', () async {
expect(await file.openRead().first, equals(bytes));
});
test('Stream can be sliced', () async {
expect(await file.openRead(2, 5).first, equals(bytes.sublist(2, 5)));
});
test('Function saveTo(..) creates file', () async {
final Directory tempDir = Directory.systemTemp.createTempSync();
final File targetFile = File('${tempDir.path}/newFilePath.txt');
if (targetFile.existsSync()) {
await targetFile.delete();
}
await file.saveTo(targetFile.path);
expect(targetFile.existsSync(), isTrue);
expect(targetFile.readAsStringSync(), 'Hello, world!');
await tempDir.delete(recursive: true);
});
});
}
// This is to create an analysis error if the version of XFile in
// interface.dart, which should never actually be used but is what the analyzer
// runs against, has the nullability of `path` changed.
XFile _ensureNonnullPathArgument(String? path) {
return XFile(path!);
}
/// An XFile subclass that tracks reads, for testing purposes.
class TestXFile extends XFile {
TestXFile(super.path);
bool hasBeenRead = false;
@override
Future<Uint8List> readAsBytes() {
hasBeenRead = true;
return super.readAsBytes();
}
}
| packages/packages/cross_file/test/x_file_io_test.dart/0 | {
"file_path": "packages/packages/cross_file/test/x_file_io_test.dart",
"repo_id": "packages",
"token_count": 1477
} | 911 |
buildFlags:
_pluginToolsConfigGlobalKey:
- "--no-tree-shake-icons"
- "--dart-define=buildmode=testing"
| packages/packages/dynamic_layouts/example/.pluginToolsConfig.yaml/0 | {
"file_path": "packages/packages/dynamic_layouts/example/.pluginToolsConfig.yaml",
"repo_id": "packages",
"token_count": 45
} | 912 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:example/staggered_layout_example.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
testWidgets('StaggeredExample lays out children correctly',
(WidgetTester tester) async {
tester.view.physicalSize = const Size(400, 200);
tester.view.devicePixelRatio = 1.0;
addTearDown(tester.view.reset);
await tester.pumpWidget(
const MaterialApp(
home: StaggeredExample(),
),
);
await tester.pumpAndSettle();
expect(find.text('Index 0'), findsOneWidget);
expect(tester.getTopLeft(find.text('Index 0')), const Offset(0.0, 56.0));
expect(find.text('Index 8'), findsOneWidget);
expect(tester.getTopLeft(find.text('Index 8')), const Offset(100.0, 146.0));
expect(find.text('Index 10'), findsOneWidget);
expect(
tester.getTopLeft(find.text('Index 10')),
const Offset(200.0, 196.0),
);
});
}
| packages/packages/dynamic_layouts/example/test/staggered_example_test.dart/0 | {
"file_path": "packages/packages/dynamic_layouts/example/test/staggered_example_test.dart",
"repo_id": "packages",
"token_count": 416
} | 913 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:dynamic_layouts/dynamic_layouts.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('DynamicSliverGridGeometry returns tight constraints for finite extents',
() {
const DynamicSliverGridGeometry geometry = DynamicSliverGridGeometry(
scrollOffset: 0,
crossAxisOffset: 0,
crossAxisExtent: 150.0,
mainAxisExtent: 50.0,
);
// Vertical
SliverConstraints sliverConstraints = const SliverConstraints(
axisDirection: AxisDirection.down,
growthDirection: GrowthDirection.forward,
userScrollDirection: ScrollDirection.forward,
scrollOffset: 0,
precedingScrollExtent: 0,
overlap: 0,
remainingPaintExtent: 600,
crossAxisExtent: 300,
crossAxisDirection: AxisDirection.left,
viewportMainAxisExtent: 1000,
remainingCacheExtent: 600,
cacheOrigin: 0.0,
);
BoxConstraints constraints = geometry.getBoxConstraints(sliverConstraints);
expect(constraints, BoxConstraints.tight(const Size(150.0, 50.0)));
// Horizontal
sliverConstraints = const SliverConstraints(
axisDirection: AxisDirection.left,
growthDirection: GrowthDirection.forward,
userScrollDirection: ScrollDirection.forward,
scrollOffset: 0,
precedingScrollExtent: 0,
overlap: 0,
remainingPaintExtent: 600,
crossAxisExtent: 300,
crossAxisDirection: AxisDirection.down,
viewportMainAxisExtent: 1000,
remainingCacheExtent: 600,
cacheOrigin: 0.0,
);
constraints = geometry.getBoxConstraints(sliverConstraints);
expect(constraints, BoxConstraints.tight(const Size(50.0, 150.0)));
});
test(
'DynamicSliverGridGeometry returns loose constraints for infinite extents',
() {
const DynamicSliverGridGeometry geometry = DynamicSliverGridGeometry(
scrollOffset: 0,
crossAxisOffset: 0,
mainAxisExtent: double.infinity,
crossAxisExtent: double.infinity,
);
// Vertical
SliverConstraints sliverConstraints = const SliverConstraints(
axisDirection: AxisDirection.down,
growthDirection: GrowthDirection.forward,
userScrollDirection: ScrollDirection.forward,
scrollOffset: 0,
precedingScrollExtent: 0,
overlap: 0,
remainingPaintExtent: 600,
crossAxisExtent: 300,
crossAxisDirection: AxisDirection.left,
viewportMainAxisExtent: 1000,
remainingCacheExtent: 600,
cacheOrigin: 0.0,
);
BoxConstraints constraints = geometry.getBoxConstraints(sliverConstraints);
expect(constraints, const BoxConstraints());
// Horizontal
sliverConstraints = const SliverConstraints(
axisDirection: AxisDirection.left,
growthDirection: GrowthDirection.forward,
userScrollDirection: ScrollDirection.forward,
scrollOffset: 0,
precedingScrollExtent: 0,
overlap: 0,
remainingPaintExtent: 600,
crossAxisExtent: 300,
crossAxisDirection: AxisDirection.down,
viewportMainAxisExtent: 1000,
remainingCacheExtent: 600,
cacheOrigin: 0.0,
);
constraints = geometry.getBoxConstraints(sliverConstraints);
expect(constraints, const BoxConstraints());
});
}
| packages/packages/dynamic_layouts/test/base_grid_layout_test.dart/0 | {
"file_path": "packages/packages/dynamic_layouts/test/base_grid_layout_test.dart",
"repo_id": "packages",
"token_count": 1317
} | 914 |
{
"name": "Google Sign In + googleapis",
"short_name": "gsi+googleapis",
"start_url": ".",
"display": "standalone",
"background_color": "#0175C2",
"theme_color": "#0175C2",
"description": "The Google Sign In example app, using the googleapis package.",
"orientation": "portrait-primary",
"prefer_related_applications": false,
"icons": [
{
"src": "icons/Icon-192.png",
"sizes": "192x192",
"type": "image/png"
},
{
"src": "icons/Icon-512.png",
"sizes": "512x512",
"type": "image/png"
},
{
"src": "icons/Icon-maskable-192.png",
"sizes": "192x192",
"type": "image/png",
"purpose": "maskable"
},
{
"src": "icons/Icon-maskable-512.png",
"sizes": "512x512",
"type": "image/png",
"purpose": "maskable"
}
]
}
| packages/packages/extension_google_sign_in_as_googleapis_auth/example/web/manifest.json/0 | {
"file_path": "packages/packages/extension_google_sign_in_as_googleapis_auth/example/web/manifest.json",
"repo_id": "packages",
"token_count": 530
} | 915 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package dev.flutter.packages.file_selector_android;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import io.flutter.embedding.engine.plugins.FlutterPlugin;
import io.flutter.embedding.engine.plugins.activity.ActivityAware;
import io.flutter.embedding.engine.plugins.activity.ActivityPluginBinding;
/** Native portion of the Android platform implementation of the file_selector plugin. */
public class FileSelectorAndroidPlugin implements FlutterPlugin, ActivityAware {
@Nullable private FileSelectorApiImpl fileSelectorApi;
private FlutterPluginBinding pluginBinding;
@Override
public void onAttachedToEngine(@NonNull FlutterPluginBinding binding) {
pluginBinding = binding;
}
@Override
public void onDetachedFromEngine(@NonNull FlutterPluginBinding binding) {
pluginBinding = null;
}
@Override
public void onAttachedToActivity(@NonNull ActivityPluginBinding binding) {
fileSelectorApi = new FileSelectorApiImpl(binding);
GeneratedFileSelectorApi.FileSelectorApi.setup(
pluginBinding.getBinaryMessenger(), fileSelectorApi);
}
@Override
public void onDetachedFromActivityForConfigChanges() {
if (fileSelectorApi != null) {
fileSelectorApi.setActivityPluginBinding(null);
}
}
@Override
public void onReattachedToActivityForConfigChanges(@NonNull ActivityPluginBinding binding) {
if (fileSelectorApi != null) {
fileSelectorApi.setActivityPluginBinding(binding);
} else {
fileSelectorApi = new FileSelectorApiImpl(binding);
GeneratedFileSelectorApi.FileSelectorApi.setup(
pluginBinding.getBinaryMessenger(), fileSelectorApi);
}
}
@Override
public void onDetachedFromActivity() {
if (fileSelectorApi != null) {
fileSelectorApi.setActivityPluginBinding(null);
}
}
}
| packages/packages/file_selector/file_selector_android/android/src/main/java/dev/flutter/packages/file_selector_android/FileSelectorAndroidPlugin.java/0 | {
"file_path": "packages/packages/file_selector/file_selector_android/android/src/main/java/dev/flutter/packages/file_selector_android/FileSelectorAndroidPlugin.java",
"repo_id": "packages",
"token_count": 642
} | 916 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:file_selector_platform_interface/file_selector_platform_interface.dart';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
/// Screen that allows the user to select an image file using
/// `openFiles`, then displays the selected images in a gallery dialog.
class OpenImagePage extends StatelessWidget {
/// Default Constructor
const OpenImagePage({super.key});
Future<void> _openImageFile(BuildContext context) async {
const XTypeGroup typeGroup = XTypeGroup(
label: 'images',
extensions: <String>['jpg', 'png'],
uniformTypeIdentifiers: <String>['public.image'],
);
final XFile? file = await FileSelectorPlatform.instance
.openFile(acceptedTypeGroups: <XTypeGroup>[typeGroup]);
if (file == null) {
// Operation was canceled by the user.
return;
}
final Uint8List bytes = await file.readAsBytes();
if (context.mounted) {
await showDialog<void>(
context: context,
builder: (BuildContext context) => ImageDisplay(file.path, bytes),
);
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Open an image'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
style: ElevatedButton.styleFrom(
foregroundColor: Colors.blue,
backgroundColor: Colors.white,
),
child: const Text('Press to open an image file(png, jpg)'),
onPressed: () => _openImageFile(context),
),
],
),
),
);
}
}
/// Widget that displays an image in a dialog.
class ImageDisplay extends StatelessWidget {
/// Default Constructor.
const ImageDisplay(this.filePath, this.bytes, {super.key});
/// The path to the selected file.
final String filePath;
/// The bytes of the selected file.
final Uint8List bytes;
@override
Widget build(BuildContext context) {
return AlertDialog(
title: Text(key: const Key('result_image_name'), filePath),
content: Image.memory(bytes),
actions: <Widget>[
TextButton(
child: const Text('Close'),
onPressed: () {
Navigator.pop(context);
},
),
],
);
}
}
| packages/packages/file_selector/file_selector_android/example/lib/open_image_page.dart/0 | {
"file_path": "packages/packages/file_selector/file_selector_android/example/lib/open_image_page.dart",
"repo_id": "packages",
"token_count": 1000
} | 917 |
## NEXT
* Updates minimum iOS version to 12.0 and minimum Flutter version to 3.16.6.
## 0.5.1+8
* Adds privacy manifest.
* Updates minimum supported SDK version to Flutter 3.10/Dart 3.0.
## 0.5.1+7
* Updates to Pigeon 13.
## 0.5.1+6
* Adds pub topics to package metadata.
* Updates minimum supported SDK version to Flutter 3.7/Dart 2.19.
## 0.5.1+5
* Fixes the behavior of no type groups to allow selecting any file.
* Migrates `styleFrom` usage in examples off of deprecated `primary` and `onPrimary` parameters.
## 0.5.1+4
* Updates references to the deprecated `macUTIs`.
## 0.5.1+3
* Updates pigeon to fix warnings with clang 15.
* Updates minimum Flutter version to 3.3.
## 0.5.1+2
* Updates to `pigeon` version 9.
## 0.5.1+1
* Clarifies explanation of endorsement in README.
* Aligns Dart and Flutter SDK constraints.
## 0.5.1
* Updates minimum Flutter version to 3.3 and iOS 11.
## 0.5.0+3
* Updates links for the merge of flutter/plugins into flutter/packages.
* Updates example code for `use_build_context_synchronously` lint.
* Updates minimum Flutter version to 3.0.
## 0.5.0+2
* Changes XTypeGroup initialization from final to const.
* Updates minimum Flutter version to 2.10.
## 0.5.0+1
* Updates README for endorsement.
## 0.5.0
* Initial iOS implementation of `file_selector`.
| packages/packages/file_selector/file_selector_ios/CHANGELOG.md/0 | {
"file_path": "packages/packages/file_selector/file_selector_ios/CHANGELOG.md",
"repo_id": "packages",
"token_count": 449
} | 918 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:file_selector_platform_interface/file_selector_platform_interface.dart';
import 'package:flutter/material.dart';
/// Screen that allows the user to select any file file using `openFile`, then
/// displays its path in a dialog.
class OpenAnyPage extends StatelessWidget {
/// Default Constructor
const OpenAnyPage({super.key});
Future<void> _openTextFile(BuildContext context) async {
final XFile? file = await FileSelectorPlatform.instance.openFile();
if (file == null) {
// Operation was canceled by the user.
return;
}
if (context.mounted) {
await showDialog<void>(
context: context,
builder: (BuildContext context) => PathDisplay(file.name, file.path),
);
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Open a file'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
style: ElevatedButton.styleFrom(
backgroundColor: Colors.blue,
foregroundColor: Colors.white,
),
child: const Text('Press to open a file of any type'),
onPressed: () => _openTextFile(context),
),
],
),
),
);
}
}
/// Widget that displays a text file in a dialog.
class PathDisplay extends StatelessWidget {
/// Default Constructor.
const PathDisplay(this.fileName, this.filePath, {super.key});
/// The name of the selected file.
final String fileName;
/// The contents of the text file.
final String filePath;
@override
Widget build(BuildContext context) {
return AlertDialog(
title: Text(fileName),
content: Text(filePath),
actions: <Widget>[
TextButton(
child: const Text('Close'),
onPressed: () => Navigator.pop(context),
),
],
);
}
}
| packages/packages/file_selector/file_selector_ios/example/lib/open_any_page.dart/0 | {
"file_path": "packages/packages/file_selector/file_selector_ios/example/lib/open_any_page.dart",
"repo_id": "packages",
"token_count": 839
} | 919 |
name: file_selector_linux_example
description: Local testbed for Linux file_selector implementation.
publish_to: 'none'
version: 1.0.0+1
environment:
sdk: ^3.1.0
flutter: ">=3.13.0"
dependencies:
file_selector_linux:
path: ../
file_selector_platform_interface: ^2.6.0
flutter:
sdk: flutter
dev_dependencies:
flutter_test:
sdk: flutter
flutter:
uses-material-design: true
| packages/packages/file_selector/file_selector_linux/example/pubspec.yaml/0 | {
"file_path": "packages/packages/file_selector/file_selector_linux/example/pubspec.yaml",
"repo_id": "packages",
"token_count": 163
} | 920 |
# file\_selector\_macos
The macOS implementation of [`file_selector`][1].
## Usage
This package is [endorsed][2], which means you can simply use `file_selector`
normally. This package will be automatically included in your app when you do,
so you do not need to add it to your `pubspec.yaml`.
However, if you `import` this package to use any of its APIs directly, you
should add it to your `pubspec.yaml` as usual.
### Entitlements
You will need to [add an entitlement][3] for either read-only access:
```xml
<key>com.apple.security.files.user-selected.read-only</key>
<true/>
```
or read/write access:
```xml
<key>com.apple.security.files.user-selected.read-write</key>
<true/>
```
depending on your use case.
[1]: https://pub.dev/packages/file_selector
[2]: https://flutter.dev/docs/development/packages-and-plugins/developing-packages#endorsed-federated-plugin
[3]: https://flutter.dev/desktop#entitlements-and-the-app-sandbox
| packages/packages/file_selector/file_selector_macos/README.md/0 | {
"file_path": "packages/packages/file_selector/file_selector_macos/README.md",
"repo_id": "packages",
"token_count": 313
} | 921 |
#include "../../Flutter/Flutter-Debug.xcconfig"
#include "Warnings.xcconfig"
| packages/packages/file_selector/file_selector_macos/example/macos/Runner/Configs/Debug.xcconfig/0 | {
"file_path": "packages/packages/file_selector/file_selector_macos/example/macos/Runner/Configs/Debug.xcconfig",
"repo_id": "packages",
"token_count": 32
} | 922 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'breakpoints.dart';
/// A Widget that takes a mapping of [SlotLayoutConfig]s to [Breakpoint]s and
/// adds the appropriate Widget based on the current screen size.
///
/// See also:
/// * [AdaptiveLayout], where [SlotLayout]s are assigned to placements on the
/// screen called "slots".
class SlotLayout extends StatefulWidget {
/// Creates a [SlotLayout] widget.
const SlotLayout({required this.config, super.key});
/// Given a context and a config, it returns the [SlotLayoutConfig] that will g
/// be chosen from the config under the context's conditions.
static SlotLayoutConfig? pickWidget(
BuildContext context, Map<Breakpoint, SlotLayoutConfig?> config) {
SlotLayoutConfig? chosenWidget;
config.forEach((Breakpoint breakpoint, SlotLayoutConfig? pickedWidget) {
if (breakpoint.isActive(context)) {
chosenWidget = pickedWidget;
}
});
return chosenWidget;
}
/// Maps [Breakpoint]s to [SlotLayoutConfig]s to determine what Widget to
/// display on which condition of screens.
///
/// The [SlotLayoutConfig]s in this map are nullable since some breakpoints
/// apply to more open ranges and the nullability allows one to override the
/// value at that Breakpoint to be null.
///
/// [SlotLayout] picks the last [SlotLayoutConfig] whose corresponding
/// [Breakpoint.isActive] returns true.
///
/// If two [Breakpoint]s are active concurrently then the latter one defined
/// in the map takes priority.
final Map<Breakpoint, SlotLayoutConfig?> config;
/// A wrapper for the children passed to [SlotLayout] to provide appropriate
/// config information.
///
/// Acts as a delegate to the abstract class [SlotLayoutConfig].
/// It first takes a builder which returns the child Widget that [SlotLayout]
/// eventually displays with an animation.
///
/// It also takes an inAnimation and outAnimation to describe how the Widget
/// should be animated as it is switched in or out from [SlotLayout]. These
/// are both defined as functions that takes a [Widget] and an [Animation] and
/// return a [Widget]. These functions are passed to the [AnimatedSwitcher]
/// inside [SlotLayout] and are to be played when the child enters/exits.
///
/// Last, it takes a required key. The key should be kept constant but unique
/// as this key is what is used to let the [SlotLayout] know that a change has
/// been made to its child.
///
/// If you define a given animation phase, there may be multiple
/// widgets being displayed depending on the phases you have chosen to animate.
/// If you are using GlobalKeys, this may cause issues with the
/// [AnimatedSwitcher].
///
/// See also:
///
/// * [AnimatedWidget] and [ImplicitlyAnimatedWidget], which are commonly used
/// as the returned widget for the inAnimation and outAnimation functions.
/// * [AnimatedSwitcher.defaultTransitionBuilder], which is what takes the
/// inAnimation and outAnimation.
static SlotLayoutConfig from({
WidgetBuilder? builder,
Widget Function(Widget, Animation<double>)? inAnimation,
Widget Function(Widget, Animation<double>)? outAnimation,
required Key key,
}) =>
SlotLayoutConfig._(
builder: builder,
inAnimation: inAnimation,
outAnimation: outAnimation,
key: key,
);
@override
State<SlotLayout> createState() => _SlotLayoutState();
}
class _SlotLayoutState extends State<SlotLayout>
with SingleTickerProviderStateMixin {
SlotLayoutConfig? chosenWidget;
@override
Widget build(BuildContext context) {
chosenWidget = SlotLayout.pickWidget(context, widget.config);
bool hasAnimation = false;
return AnimatedSwitcher(
duration: const Duration(milliseconds: 1000),
layoutBuilder: (Widget? currentChild, List<Widget> previousChildren) {
final Stack elements = Stack(
children: <Widget>[
if (hasAnimation && previousChildren.isNotEmpty)
previousChildren.first,
if (currentChild != null) currentChild,
],
);
return elements;
},
transitionBuilder: (Widget child, Animation<double> animation) {
final SlotLayoutConfig configChild = child as SlotLayoutConfig;
if (child.key == chosenWidget?.key) {
return (configChild.inAnimation != null)
? child.inAnimation!(child, animation)
: child;
} else {
if (configChild.outAnimation != null) {
hasAnimation = true;
}
return (configChild.outAnimation != null)
? child.outAnimation!(child, ReverseAnimation(animation))
: child;
}
},
child: chosenWidget ?? SlotLayoutConfig.empty());
}
}
/// Defines how [SlotLayout] should display under a certain [Breakpoint].
class SlotLayoutConfig extends StatelessWidget {
/// Creates a new [SlotLayoutConfig].
///
/// Returns the child widget as is but holds properties to be accessed by other
/// classes.
const SlotLayoutConfig._({
super.key,
required this.builder,
this.inAnimation,
this.outAnimation,
});
/// The child Widget that [SlotLayout] eventually returns with an animation.
final WidgetBuilder? builder;
/// A function that provides the animation to be wrapped around the builder
/// child as it is being moved in during a switch in [SlotLayout].
///
/// See also:
///
/// * [AnimatedWidget] and [ImplicitlyAnimatedWidget], which are commonly used
/// as the returned widget.
final Widget Function(Widget, Animation<double>)? inAnimation;
/// A function that provides the animation to be wrapped around the builder
/// child as it is being moved in during a switch in [SlotLayout].
///
/// See also:
///
/// * [AnimatedWidget] and [ImplicitlyAnimatedWidget], which are commonly used
/// as the returned widget.
final Widget Function(Widget, Animation<double>)? outAnimation;
/// An empty [SlotLayoutConfig] to be placed in a slot to indicate that the slot
/// should show nothing.
static SlotLayoutConfig empty() {
return const SlotLayoutConfig._(key: Key(''), builder: null);
}
@override
Widget build(BuildContext context) {
return (builder != null) ? builder!(context) : const SizedBox.shrink();
}
}
| packages/packages/flutter_adaptive_scaffold/lib/src/slot_layout.dart/0 | {
"file_path": "packages/packages/flutter_adaptive_scaffold/lib/src/slot_layout.dart",
"repo_id": "packages",
"token_count": 2060
} | 923 |
def localProperties = new Properties()
def localPropertiesFile = rootProject.file('local.properties')
if (localPropertiesFile.exists()) {
localPropertiesFile.withReader('UTF-8') { reader ->
localProperties.load(reader)
}
}
def flutterRoot = localProperties.getProperty('flutter.sdk')
if (flutterRoot == null) {
throw new GradleException("Flutter SDK not found. Define location with flutter.sdk in the local.properties file.")
}
def flutterVersionCode = localProperties.getProperty('flutter.versionCode')
if (flutterVersionCode == null) {
flutterVersionCode = '1'
}
def flutterVersionName = localProperties.getProperty('flutter.versionName')
if (flutterVersionName == null) {
flutterVersionName = '1.0'
}
apply plugin: 'com.android.application'
apply plugin: 'kotlin-android'
apply from: "$flutterRoot/packages/flutter_tools/gradle/flutter.gradle"
android {
namespace "com.example.example"
compileSdk flutter.compileSdkVersion
ndkVersion flutter.ndkVersion
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
kotlinOptions {
jvmTarget = '1.8'
}
sourceSets {
main.java.srcDirs += 'src/main/kotlin'
}
defaultConfig {
applicationId "dev.flutter.packages.flutterimageexample"
// You can update the following values to match your application needs.
// For more information, see: https://docs.flutter.dev/deployment/android#reviewing-the-gradle-build-configuration.
minSdkVersion flutter.minSdkVersion
targetSdkVersion flutter.targetSdkVersion
versionCode flutterVersionCode.toInteger()
versionName flutterVersionName
}
buildTypes {
release {
signingConfig signingConfigs.debug
}
}
}
flutter {
source '../..'
}
dependencies {
implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk7:$kotlin_version"
}
| packages/packages/flutter_image/example/android/app/build.gradle/0 | {
"file_path": "packages/packages/flutter_image/example/android/app/build.gradle",
"repo_id": "packages",
"token_count": 726
} | 924 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/widgets.dart';
import 'package:flutter_image/flutter_image.dart';
import 'package:flutter_image_example/readme_excerpts.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test('networkImageWithRetry returns an Image with NetworkImageWithRetry', () {
// Ensure that the snippet code runs successfully.
final Image result = networkImageWithRetry();
// It should have a image property of the right type.
expect(result.image, isInstanceOf<NetworkImageWithRetry>());
// And the NetworkImageWithRetry should have a url property.
final NetworkImageWithRetry networkImage =
result.image as NetworkImageWithRetry;
expect(networkImage.url, equals('http://example.com/avatars/123.jpg'));
});
}
| packages/packages/flutter_image/example/test/readme_excerpts_test.dart/0 | {
"file_path": "packages/packages/flutter_image/example/test/readme_excerpts_test.dart",
"repo_id": "packages",
"token_count": 286
} | 925 |
buildFlags:
_pluginToolsConfigGlobalKey:
- "--no-tree-shake-icons"
- "--dart-define=buildmode=testing"
| packages/packages/flutter_markdown/example/.pluginToolsConfig.yaml/0 | {
"file_path": "packages/packages/flutter_markdown/example/.pluginToolsConfig.yaml",
"repo_id": "packages",
"token_count": 45
} | 926 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_markdown/flutter_markdown.dart';
import 'package:markdown/markdown.dart' as md;
import '../shared/markdown_demo_widget.dart';
import '../shared/markdown_extensions.dart';
// ignore_for_file: public_member_api_docs
const String _notes = """
# Extended Emoji Demo
---
## Overview
This simple example demonstrates how to subclass an existing inline syntax
parser to extend, enhance, or modify its behavior. This example shows how to
subclass the EmojiSyntax inline syntax parser to support alternative names for
the thumbs up and thumbs down emoji characters. The emoji character map used by
EmojiSyntax has the keys "+1" and "-1" associated with the thumbs up and thumbs
down emoji characters, respectively. The ExtendedEmojiSyntax subclass extends
the EmojiSyntax class by overriding the onMatch method to intercept the call
from the parser. ExtendedEmojiSyntax either handles the matched tag or passes
the match along to its parent for processing.
```
class ExtendedEmojiSyntax extends md.EmojiSyntax {
static const alternateTags = <String, String>{
'thumbsup': '👍',
'thumbsdown': '👎',
};
@override
bool onMatch(md.InlineParser parser, Match match) {
var emoji = alternateTags[match[1]];
if (emoji != null) {
parser.addNode(md.Text(emoji));
return true;
}
return super.onMatch(parser, match);
}
}
```
""";
// TODO(goderbauer): Restructure the examples to avoid this ignore, https://github.com/flutter/flutter/issues/110208.
// ignore: avoid_implementing_value_types
class ExtendedEmojiDemo extends StatelessWidget implements MarkdownDemoWidget {
const ExtendedEmojiDemo({super.key});
static const String _title = 'Extended Emoji Demo';
@override
String get title => ExtendedEmojiDemo._title;
@override
String get description => 'Demonstrates how to extend an existing inline'
' syntax parser by intercepting the parser onMatch routine.';
@override
Future<String> get data =>
Future<String>.value('Simple test :smiley: :thumbsup:!');
@override
Future<String> get notes => Future<String>.value(_notes);
static const String _notExtended = '# Using Emoji Syntax\n';
static const String _extended = '# Using Extened Emoji Syntax\n';
@override
Widget build(BuildContext context) {
return FutureBuilder<String>(
future: data,
builder: (BuildContext context, AsyncSnapshot<String> snapshot) {
if (snapshot.connectionState == ConnectionState.done) {
return Container(
margin: const EdgeInsets.all(12),
constraints: const BoxConstraints.expand(),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
MarkdownBody(
data: _notExtended + snapshot.data!,
extensionSet: MarkdownExtensionSet.githubWeb.value,
),
const SizedBox(
height: 24,
),
MarkdownBody(
data: _extended + snapshot.data!,
extensionSet: md.ExtensionSet(<md.BlockSyntax>[],
<md.InlineSyntax>[ExtendedEmojiSyntax()]),
),
],
),
);
} else {
return const CircularProgressIndicator();
}
},
);
}
}
class ExtendedEmojiSyntax extends md.EmojiSyntax {
static const Map<String, String> alternateTags = <String, String>{
'thumbsup': '👍',
'thumbsdown': '👎',
};
@override
bool onMatch(md.InlineParser parser, Match match) {
final String? emoji = alternateTags[match[1]!];
if (emoji != null) {
parser.addNode(md.Text(emoji));
return true;
}
return super.onMatch(parser, match);
}
}
| packages/packages/flutter_markdown/example/lib/demos/extended_emoji_demo.dart/0 | {
"file_path": "packages/packages/flutter_markdown/example/lib/demos/extended_emoji_demo.dart",
"repo_id": "packages",
"token_count": 1499
} | 927 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter_markdown/flutter_markdown.dart';
import 'package:flutter_test/flutter_test.dart';
import 'utils.dart';
void main() => defineTests();
void defineTests() {
group('Padding builders', () {
testWidgets(
'use paddingBuilders for p',
(WidgetTester tester) async {
const double paddingX = 10.0;
await tester.pumpWidget(
boilerplate(
Markdown(
data: '**line 1**\n\n# H1\n',
paddingBuilders: <String, MarkdownPaddingBuilder>{
'p': CustomPaddingBuilder(paddingX * 1),
'strong': CustomPaddingBuilder(paddingX * 2),
'h1': CustomPaddingBuilder(paddingX * 3),
'img': CustomPaddingBuilder(paddingX * 4),
}),
),
);
final List<Padding> paddings =
tester.widgetList<Padding>(find.byType(Padding)).toList();
expect(paddings.length, 4);
expect(
paddings[0].padding.along(Axis.horizontal) == paddingX * 1 * 2,
true,
);
expect(
paddings[1].padding.along(Axis.horizontal) == paddingX * 3 * 2,
true,
);
expect(
paddings[2].padding.along(Axis.horizontal) == paddingX * 1 * 2,
true,
);
expect(
paddings[3].padding.along(Axis.horizontal) == paddingX * 4 * 2,
true,
);
},
);
});
}
class CustomPaddingBuilder extends MarkdownPaddingBuilder {
CustomPaddingBuilder(this.paddingX);
double paddingX;
@override
EdgeInsets getPadding() {
return EdgeInsets.symmetric(horizontal: paddingX);
}
}
| packages/packages/flutter_markdown/test/padding_test.dart/0 | {
"file_path": "packages/packages/flutter_markdown/test/padding_test.dart",
"repo_id": "packages",
"token_count": 894
} | 928 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:process/process.dart';
import '../base/command.dart';
import '../base/file_system.dart';
import '../base/logger.dart';
import '../base/project.dart';
import '../base/terminal.dart';
import '../manifest.dart';
import '../utils.dart';
/// Flutter migrate subcommand to check the migration status of the project.
class MigrateStatusCommand extends MigrateCommand {
MigrateStatusCommand({
bool verbose = false,
required this.logger,
required this.fileSystem,
required ProcessManager processManager,
this.standalone = false,
}) : _verbose = verbose,
migrateUtils = MigrateUtils(
logger: logger,
fileSystem: fileSystem,
processManager: processManager,
) {
argParser.addOption(
'staging-directory',
help: 'Specifies the custom migration working directory used to stage '
'and edit proposed changes. This path can be absolute or relative '
'to the flutter project root. This defaults to '
'`$kDefaultMigrateStagingDirectoryName`',
valueHelp: 'path',
);
argParser.addOption(
'project-directory',
help: 'The root directory of the flutter project. This defaults to the '
'current working directory if omitted.',
valueHelp: 'path',
);
argParser.addFlag(
'diff',
defaultsTo: true,
help: 'Shows the diff output when enabled. Enabled by default.',
);
argParser.addFlag(
'show-added-files',
help: 'Shows the contents of added files. Disabled by default.',
);
argParser.addFlag(
'flutter-subcommand',
help:
'Enable when using the flutter tool as a subcommand. This changes the '
'wording of log messages to indicate the correct suggested commands to use.',
);
}
final bool _verbose;
final Logger logger;
final FileSystem fileSystem;
final MigrateUtils migrateUtils;
final bool standalone;
@override
final String name = 'status';
@override
final String description =
'Prints the current status of the in progress migration.';
/// Manually marks the lines in a diff that should be printed unformatted for visbility.
///
/// This is used to ensure the initial lines that display the files being diffed and the
/// git revisions are printed and never skipped.
final Set<int> _initialDiffLines = <int>{0, 1};
@override
Future<CommandResult> runCommand() async {
final String? projectDirectory = stringArg('project-directory');
final FlutterProjectFactory flutterProjectFactory = FlutterProjectFactory();
final FlutterProject project = projectDirectory == null
? FlutterProject.current(fileSystem)
: flutterProjectFactory
.fromDirectory(fileSystem.directory(projectDirectory));
final bool isSubcommand = boolArg('flutter-subcommand') ?? !standalone;
if (!validateWorkingDirectory(project, logger)) {
return CommandResult.fail();
}
Directory stagingDirectory =
project.directory.childDirectory(kDefaultMigrateStagingDirectoryName);
final String? customStagingDirectoryPath = stringArg('staging-directory');
if (customStagingDirectoryPath != null) {
if (fileSystem.path.isAbsolute(customStagingDirectoryPath)) {
stagingDirectory = fileSystem.directory(customStagingDirectoryPath);
} else {
stagingDirectory =
project.directory.childDirectory(customStagingDirectoryPath);
}
}
if (!stagingDirectory.existsSync()) {
logger.printStatus(
'No migration in progress in $stagingDirectory. Start a new migration with:');
printCommandText('start', logger, standalone: !isSubcommand);
return const CommandResult(ExitStatus.fail);
}
final File manifestFile =
MigrateManifest.getManifestFileFromDirectory(stagingDirectory);
if (!manifestFile.existsSync()) {
logger.printError('No migrate manifest in the migrate working directory '
'at ${stagingDirectory.path}. Fix the working directory '
'or abandon and restart the migration.');
return const CommandResult(ExitStatus.fail);
}
final MigrateManifest manifest = MigrateManifest.fromFile(manifestFile);
final bool showDiff = boolArg('diff') ?? true;
final bool showAddedFiles = boolArg('show-added-files') ?? true;
if (showDiff || _verbose) {
if (showAddedFiles || _verbose) {
for (final String localPath in manifest.addedFiles) {
logger.printStatus('Newly added file at $localPath:\n');
try {
logger.printStatus(
stagingDirectory.childFile(localPath).readAsStringSync(),
color: TerminalColor.green);
} on FileSystemException {
logger.printStatus('Contents are byte data\n',
color: TerminalColor.grey);
}
}
}
final List<String> files = <String>[];
files.addAll(manifest.mergedFiles);
files.addAll(manifest.resolvedConflictFiles(stagingDirectory));
files.addAll(manifest.remainingConflictFiles(stagingDirectory));
for (final String localPath in files) {
final DiffResult result = await migrateUtils.diffFiles(
project.directory.childFile(localPath),
stagingDirectory.childFile(localPath));
if (result.diff != '' && result.diff != null) {
// Print with different colors for better visibility.
int lineNumber = -1;
for (final String line in result.diff!.split('\n')) {
lineNumber++;
if (line.startsWith('---') ||
line.startsWith('+++') ||
line.startsWith('&&') ||
_initialDiffLines.contains(lineNumber)) {
logger.printStatus(line);
continue;
}
if (line.startsWith('-')) {
logger.printStatus(line, color: TerminalColor.red);
continue;
}
if (line.startsWith('+')) {
logger.printStatus(line, color: TerminalColor.green);
continue;
}
logger.printStatus(line, color: TerminalColor.grey);
}
}
}
}
logger.printBox('Staging directory at `${stagingDirectory.path}`');
checkAndPrintMigrateStatus(manifest, stagingDirectory, logger: logger);
final bool readyToApply =
manifest.remainingConflictFiles(stagingDirectory).isEmpty;
if (!readyToApply) {
logger.printStatus('Guided conflict resolution wizard:');
printCommandText('resolve-conflicts', logger, standalone: !isSubcommand);
logger.printStatus('Resolve conflicts and accept changes with:');
} else {
logger.printStatus(
'All conflicts resolved. Review changes above and '
'apply the migration with:',
color: TerminalColor.green);
}
printCommandText('apply', logger, standalone: !isSubcommand);
return const CommandResult(ExitStatus.success);
}
}
| packages/packages/flutter_migrate/lib/src/commands/status.dart/0 | {
"file_path": "packages/packages/flutter_migrate/lib/src/commands/status.dart",
"repo_id": "packages",
"token_count": 2664
} | 929 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter_migrate/src/base/io.dart';
import 'package:flutter_migrate/src/base/logger.dart';
import 'package:flutter_migrate/src/base/terminal.dart';
import 'package:test/fake.dart';
import '../src/common.dart';
import '../src/fakes.dart';
void main() {
testWithoutContext('correct logger instance is created', () {
final LoggerFactory loggerFactory = LoggerFactory(
terminal: Terminal.test(),
stdio: FakeStdio(),
outputPreferences: OutputPreferences.test(),
);
expect(
loggerFactory.createLogger(
windows: false,
),
isA<StdoutLogger>());
expect(
loggerFactory.createLogger(
windows: true,
),
isA<WindowsStdoutLogger>());
});
testWithoutContext(
'WindowsStdoutLogger rewrites emojis when terminal does not support emoji',
() {
final FakeStdio stdio = FakeStdio();
final WindowsStdoutLogger logger = WindowsStdoutLogger(
outputPreferences: OutputPreferences.test(),
stdio: stdio,
terminal: Terminal.test(),
);
logger.printStatus('🔥🖼️✗✓🔨💪✏️');
expect(stdio.writtenToStdout, <String>['X√\n']);
});
testWithoutContext(
'WindowsStdoutLogger does not rewrite emojis when terminal does support emoji',
() {
final FakeStdio stdio = FakeStdio();
final WindowsStdoutLogger logger = WindowsStdoutLogger(
outputPreferences: OutputPreferences.test(),
stdio: stdio,
terminal: Terminal.test(supportsColor: true, supportsEmoji: true),
);
logger.printStatus('🔥🖼️✗✓🔨💪✏️');
expect(stdio.writtenToStdout, <String>['🔥🖼️✗✓🔨💪✏️\n']);
});
testWithoutContext(
'Logger does not throw when stdio write throws synchronously', () async {
final FakeStdout stdout = FakeStdout(syncError: true);
final FakeStdout stderr = FakeStdout(syncError: true);
final Stdio stdio = Stdio.test(stdout: stdout, stderr: stderr);
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: stdio,
),
stdio: stdio,
outputPreferences: OutputPreferences.test(),
);
logger.printStatus('message');
logger.printError('error message');
});
testWithoutContext(
'Logger does not throw when stdio write throws asynchronously', () async {
final FakeStdout stdout = FakeStdout(syncError: false);
final FakeStdout stderr = FakeStdout(syncError: false);
final Stdio stdio = Stdio.test(stdout: stdout, stderr: stderr);
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: stdio,
),
stdio: stdio,
outputPreferences: OutputPreferences.test(),
);
logger.printStatus('message');
logger.printError('error message');
await stdout.done;
await stderr.done;
});
testWithoutContext(
'Logger does not throw when stdio completes done with an error',
() async {
final FakeStdout stdout =
FakeStdout(syncError: false, completeWithError: true);
final FakeStdout stderr =
FakeStdout(syncError: false, completeWithError: true);
final Stdio stdio = Stdio.test(stdout: stdout, stderr: stderr);
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: stdio,
),
stdio: stdio,
outputPreferences: OutputPreferences.test(),
);
logger.printStatus('message');
logger.printError('error message');
expect(() async => stdout.done, throwsException);
expect(() async => stderr.done, throwsException);
});
group('Output format', () {
late FakeStdio fakeStdio;
late SummaryStatus summaryStatus;
late int called;
setUp(() {
fakeStdio = FakeStdio();
called = 0;
summaryStatus = SummaryStatus(
message: 'Hello world',
padding: 20,
onFinish: () => called++,
stdio: fakeStdio,
stopwatch: FakeStopwatch(),
);
});
List<String> outputStdout() => fakeStdio.writtenToStdout.join().split('\n');
List<String> outputStderr() => fakeStdio.writtenToStderr.join().split('\n');
testWithoutContext('Error logs are wrapped', () async {
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: fakeStdio,
),
stdio: fakeStdio,
outputPreferences:
OutputPreferences.test(wrapText: true, wrapColumn: 40),
);
logger.printError('0123456789' * 15);
final List<String> lines = outputStderr();
expect(outputStdout().length, equals(1));
expect(outputStdout().first, isEmpty);
expect(lines[0], equals('0123456789' * 4));
expect(lines[1], equals('0123456789' * 4));
expect(lines[2], equals('0123456789' * 4));
expect(lines[3], equals('0123456789' * 3));
});
testWithoutContext('Error logs are wrapped and can be indented.', () async {
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: fakeStdio,
),
stdio: fakeStdio,
outputPreferences:
OutputPreferences.test(wrapText: true, wrapColumn: 40),
);
logger.printError('0123456789' * 15, indent: 5);
final List<String> lines = outputStderr();
expect(outputStdout().length, equals(1));
expect(outputStdout().first, isEmpty);
expect(lines.length, equals(6));
expect(lines[0], equals(' 01234567890123456789012345678901234'));
expect(lines[1], equals(' 56789012345678901234567890123456789'));
expect(lines[2], equals(' 01234567890123456789012345678901234'));
expect(lines[3], equals(' 56789012345678901234567890123456789'));
expect(lines[4], equals(' 0123456789'));
expect(lines[5], isEmpty);
});
testWithoutContext('Error logs are wrapped and can have hanging indent.',
() async {
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: fakeStdio,
),
stdio: fakeStdio,
outputPreferences:
OutputPreferences.test(wrapText: true, wrapColumn: 40),
);
logger.printError('0123456789' * 15, hangingIndent: 5);
final List<String> lines = outputStderr();
expect(outputStdout().length, equals(1));
expect(outputStdout().first, isEmpty);
expect(lines.length, equals(6));
expect(lines[0], equals('0123456789012345678901234567890123456789'));
expect(lines[1], equals(' 01234567890123456789012345678901234'));
expect(lines[2], equals(' 56789012345678901234567890123456789'));
expect(lines[3], equals(' 01234567890123456789012345678901234'));
expect(lines[4], equals(' 56789'));
expect(lines[5], isEmpty);
});
testWithoutContext(
'Error logs are wrapped, indented, and can have hanging indent.',
() async {
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: fakeStdio,
),
stdio: fakeStdio,
outputPreferences:
OutputPreferences.test(wrapText: true, wrapColumn: 40),
);
logger.printError('0123456789' * 15, indent: 4, hangingIndent: 5);
final List<String> lines = outputStderr();
expect(outputStdout().length, equals(1));
expect(outputStdout().first, isEmpty);
expect(lines.length, equals(6));
expect(lines[0], equals(' 012345678901234567890123456789012345'));
expect(lines[1], equals(' 6789012345678901234567890123456'));
expect(lines[2], equals(' 7890123456789012345678901234567'));
expect(lines[3], equals(' 8901234567890123456789012345678'));
expect(lines[4], equals(' 901234567890123456789'));
expect(lines[5], isEmpty);
});
testWithoutContext('Stdout logs are wrapped', () async {
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: fakeStdio,
),
stdio: fakeStdio,
outputPreferences:
OutputPreferences.test(wrapText: true, wrapColumn: 40),
);
logger.printStatus('0123456789' * 15);
final List<String> lines = outputStdout();
expect(outputStderr().length, equals(1));
expect(outputStderr().first, isEmpty);
expect(lines[0], equals('0123456789' * 4));
expect(lines[1], equals('0123456789' * 4));
expect(lines[2], equals('0123456789' * 4));
expect(lines[3], equals('0123456789' * 3));
});
testWithoutContext('Stdout logs are wrapped and can be indented.',
() async {
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: fakeStdio,
),
stdio: fakeStdio,
outputPreferences:
OutputPreferences.test(wrapText: true, wrapColumn: 40),
);
logger.printStatus('0123456789' * 15, indent: 5);
final List<String> lines = outputStdout();
expect(outputStderr().length, equals(1));
expect(outputStderr().first, isEmpty);
expect(lines.length, equals(6));
expect(lines[0], equals(' 01234567890123456789012345678901234'));
expect(lines[1], equals(' 56789012345678901234567890123456789'));
expect(lines[2], equals(' 01234567890123456789012345678901234'));
expect(lines[3], equals(' 56789012345678901234567890123456789'));
expect(lines[4], equals(' 0123456789'));
expect(lines[5], isEmpty);
});
testWithoutContext('Stdout logs are wrapped and can have hanging indent.',
() async {
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: fakeStdio,
),
stdio: fakeStdio,
outputPreferences:
OutputPreferences.test(wrapText: true, wrapColumn: 40));
logger.printStatus('0123456789' * 15, hangingIndent: 5);
final List<String> lines = outputStdout();
expect(outputStderr().length, equals(1));
expect(outputStderr().first, isEmpty);
expect(lines.length, equals(6));
expect(lines[0], equals('0123456789012345678901234567890123456789'));
expect(lines[1], equals(' 01234567890123456789012345678901234'));
expect(lines[2], equals(' 56789012345678901234567890123456789'));
expect(lines[3], equals(' 01234567890123456789012345678901234'));
expect(lines[4], equals(' 56789'));
expect(lines[5], isEmpty);
});
testWithoutContext(
'Stdout logs are wrapped, indented, and can have hanging indent.',
() async {
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: fakeStdio,
),
stdio: fakeStdio,
outputPreferences:
OutputPreferences.test(wrapText: true, wrapColumn: 40),
);
logger.printStatus('0123456789' * 15, indent: 4, hangingIndent: 5);
final List<String> lines = outputStdout();
expect(outputStderr().length, equals(1));
expect(outputStderr().first, isEmpty);
expect(lines.length, equals(6));
expect(lines[0], equals(' 012345678901234567890123456789012345'));
expect(lines[1], equals(' 6789012345678901234567890123456'));
expect(lines[2], equals(' 7890123456789012345678901234567'));
expect(lines[3], equals(' 8901234567890123456789012345678'));
expect(lines[4], equals(' 901234567890123456789'));
expect(lines[5], isEmpty);
});
testWithoutContext('Error logs are red', () async {
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: fakeStdio,
supportsColor: true,
),
stdio: fakeStdio,
outputPreferences: OutputPreferences.test(showColor: true),
);
logger.printError('Pants on fire!');
final List<String> lines = outputStderr();
expect(outputStdout().length, equals(1));
expect(outputStdout().first, isEmpty);
expect(
lines[0],
equals(
'${AnsiTerminal.red}Pants on fire!${AnsiTerminal.resetColor}'));
});
testWithoutContext('Stdout logs are not colored', () async {
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: fakeStdio,
),
stdio: fakeStdio,
outputPreferences: OutputPreferences.test(showColor: true),
);
logger.printStatus('All good.');
final List<String> lines = outputStdout();
expect(outputStderr().length, equals(1));
expect(outputStderr().first, isEmpty);
expect(lines[0], equals('All good.'));
});
testWithoutContext('Stdout printBox puts content inside a box', () {
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: fakeStdio,
),
stdio: fakeStdio,
outputPreferences: OutputPreferences.test(showColor: true),
);
logger.printBox('Hello world', title: 'Test title');
final String stdout = fakeStdio.writtenToStdout.join();
expect(
stdout,
contains('\n'
'┌─ Test title ┐\n'
'│ Hello world │\n'
'└─────────────┘\n'),
);
});
testWithoutContext('Stdout printBox does not require title', () {
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: fakeStdio,
),
stdio: fakeStdio,
outputPreferences: OutputPreferences.test(showColor: true),
);
logger.printBox('Hello world');
final String stdout = fakeStdio.writtenToStdout.join();
expect(
stdout,
contains('\n'
'┌─────────────┐\n'
'│ Hello world │\n'
'└─────────────┘\n'),
);
});
testWithoutContext('Stdout printBox handles new lines', () {
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: fakeStdio,
),
stdio: fakeStdio,
outputPreferences: OutputPreferences.test(showColor: true),
);
logger.printBox('Hello world\nThis is a new line', title: 'Test title');
final String stdout = fakeStdio.writtenToStdout.join();
expect(
stdout,
contains('\n'
'┌─ Test title ───────┐\n'
'│ Hello world │\n'
'│ This is a new line │\n'
'└────────────────────┘\n'),
);
});
testWithoutContext(
'Stdout printBox handles content with ANSI escape characters', () {
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: fakeStdio,
),
stdio: fakeStdio,
outputPreferences: OutputPreferences.test(showColor: true),
);
const String bold = '\u001B[1m';
const String clear = '\u001B[2J\u001B[H';
logger.printBox('${bold}Hello world$clear', title: 'Test title');
final String stdout = fakeStdio.writtenToStdout.join();
expect(
stdout,
contains('\n'
'┌─ Test title ┐\n'
'│ ${bold}Hello world$clear │\n'
'└─────────────┘\n'),
);
});
testWithoutContext('Stdout printBox handles column limit', () {
const int columnLimit = 14;
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: fakeStdio,
),
stdio: fakeStdio,
outputPreferences:
OutputPreferences.test(showColor: true, wrapColumn: columnLimit),
);
logger.printBox('This line is longer than $columnLimit characters',
title: 'Test');
final String stdout = fakeStdio.writtenToStdout.join();
final List<String> stdoutLines = stdout.split('\n');
expect(stdoutLines.length, greaterThan(1));
expect(stdoutLines[1].length, equals(columnLimit));
expect(
stdout,
contains('\n'
'┌─ Test ─────┐\n'
'│ This line │\n'
'│ is longer │\n'
'│ than 14 │\n'
'│ characters │\n'
'└────────────┘\n'),
);
});
testWithoutContext(
'Stdout printBox handles column limit and respects new lines', () {
const int columnLimit = 14;
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: fakeStdio,
),
stdio: fakeStdio,
outputPreferences:
OutputPreferences.test(showColor: true, wrapColumn: columnLimit),
);
logger.printBox('This\nline is longer than\n\n$columnLimit characters',
title: 'Test');
final String stdout = fakeStdio.writtenToStdout.join();
final List<String> stdoutLines = stdout.split('\n');
expect(stdoutLines.length, greaterThan(1));
expect(stdoutLines[1].length, equals(columnLimit));
expect(
stdout,
contains('\n'
'┌─ Test ─────┐\n'
'│ This │\n'
'│ line is │\n'
'│ longer │\n'
'│ than │\n'
'│ │\n'
'│ 14 │\n'
'│ characters │\n'
'└────────────┘\n'),
);
});
testWithoutContext(
'Stdout printBox breaks long words that exceed the column limit', () {
const int columnLimit = 14;
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: fakeStdio,
),
stdio: fakeStdio,
outputPreferences:
OutputPreferences.test(showColor: true, wrapColumn: columnLimit),
);
logger.printBox('Thiswordislongerthan${columnLimit}characters',
title: 'Test');
final String stdout = fakeStdio.writtenToStdout.join();
final List<String> stdoutLines = stdout.split('\n');
expect(stdoutLines.length, greaterThan(1));
expect(stdoutLines[1].length, equals(columnLimit));
expect(
stdout,
contains('\n'
'┌─ Test ─────┐\n'
'│ Thiswordis │\n'
'│ longerthan │\n'
'│ 14characte │\n'
'│ rs │\n'
'└────────────┘\n'),
);
});
testWithoutContext('Stdout startProgress on non-color terminal', () async {
final FakeStopwatch fakeStopwatch = FakeStopwatch();
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: fakeStdio,
),
stdio: fakeStdio,
outputPreferences: OutputPreferences.test(),
stopwatchFactory: FakeStopwatchFactory(stopwatch: fakeStopwatch),
);
final Status status = logger.startProgress(
'Hello',
progressIndicatorPadding:
20, // this minus the "Hello" equals the 15 below.
);
expect(outputStderr().length, equals(1));
expect(outputStderr().first, isEmpty);
// the 5 below is the margin that is always included between the message and the time.
expect(outputStdout().join('\n'), matches(r'^Hello {15} {5}$'));
fakeStopwatch.elapsed = const Duration(seconds: 4, milliseconds: 123);
status.stop();
expect(outputStdout(), <String>['Hello 4.1s', '']);
});
testWithoutContext('SummaryStatus works when canceled', () async {
final SummaryStatus summaryStatus = SummaryStatus(
message: 'Hello world',
padding: 20,
onFinish: () => called++,
stdio: fakeStdio,
stopwatch: FakeStopwatch(),
);
summaryStatus.start();
final List<String> lines = outputStdout();
expect(lines[0], startsWith('Hello world '));
expect(lines.length, equals(1));
expect(lines[0].endsWith('\n'), isFalse);
// Verify a cancel does _not_ print the time and prints a newline.
summaryStatus.cancel();
expect(outputStdout(), <String>[
'Hello world ',
'',
]);
// Verify that stopping or canceling multiple times throws.
expect(summaryStatus.cancel, throwsAssertionError);
expect(summaryStatus.stop, throwsAssertionError);
});
testWithoutContext('SummaryStatus works when stopped', () async {
summaryStatus.start();
final List<String> lines = outputStdout();
expect(lines[0], startsWith('Hello world '));
expect(lines.length, equals(1));
// Verify a stop prints the time.
summaryStatus.stop();
expect(outputStdout(), <String>[
'Hello world 0ms',
'',
]);
// Verify that stopping or canceling multiple times throws.
expect(summaryStatus.stop, throwsAssertionError);
expect(summaryStatus.cancel, throwsAssertionError);
});
testWithoutContext('sequential startProgress calls with StdoutLogger',
() async {
final Logger logger = StdoutLogger(
terminal: AnsiTerminal(
stdio: fakeStdio,
),
stdio: fakeStdio,
outputPreferences: OutputPreferences.test(),
);
logger.startProgress('AAA').stop();
logger.startProgress('BBB').stop();
final List<String> output = outputStdout();
expect(output.length, equals(3));
// There's 61 spaces at the start: 59 (padding default) - 3 (length of AAA) + 5 (margin).
// Then there's a left-padded "0ms" 8 characters wide, so 5 spaces then "0ms"
// (except sometimes it's randomly slow so we handle up to "99,999ms").
expect(output[0], matches(RegExp(r'AAA[ ]{61}[\d, ]{5}[\d]ms')));
expect(output[1], matches(RegExp(r'BBB[ ]{61}[\d, ]{5}[\d]ms')));
});
testWithoutContext('sequential startProgress calls with BufferLogger',
() async {
final BufferLogger logger = BufferLogger(
terminal: AnsiTerminal(
stdio: fakeStdio,
),
outputPreferences: OutputPreferences.test(),
);
logger.startProgress('AAA').stop();
logger.startProgress('BBB').stop();
expect(logger.statusText, 'AAA\nBBB\n');
});
});
}
class FakeStdout extends Fake implements Stdout {
FakeStdout({required this.syncError, this.completeWithError = false});
final bool syncError;
final bool completeWithError;
final Completer<void> _completer = Completer<void>();
@override
void write(Object? object) {
if (syncError) {
throw Exception('Error!');
}
Zone.current.runUnaryGuarded<void>((_) {
if (completeWithError) {
_completer.completeError(Exception('Some pipe error'));
} else {
_completer.complete();
throw Exception('Error!');
}
}, null);
}
@override
Future<void> get done => _completer.future;
}
| packages/packages/flutter_migrate/test/base/logger_test.dart/0 | {
"file_path": "packages/packages/flutter_migrate/test/base/logger_test.dart",
"repo_id": "packages",
"token_count": 10124
} | 930 |
flutter/test/full_app_fixtures/vanilla version:1.22.6_stable
| packages/packages/flutter_migrate/test/test_data/full_apps/vanilla_app_1_22_6_stable.ensure/0 | {
"file_path": "packages/packages/flutter_migrate/test/test_data/full_apps/vanilla_app_1_22_6_stable.ensure",
"repo_id": "packages",
"token_count": 25
} | 931 |
Instead of navigating to a route based on the URL, a GoRoute can be given a unique
name. To configure a named route, use the `name` parameter:
```dart
GoRoute(
name: 'song',
path: 'songs/:songId',
builder: /* ... */,
),
```
To navigate to a route using its name, call [`goNamed`](https://pub.dev/documentation/go_router/latest/go_router/GoRouter/goNamed.html):
```dart
TextButton(
onPressed: () {
context.goNamed('song', pathParameters: {'songId': 123});
},
child: const Text('Go to song 2'),
),
```
Alternatively, you can look up the location for a name using `namedLocation`:
```dart
TextButton(
onPressed: () {
final String location = context.namedLocation('song', pathParameters: {'songId': 123});
context.go(location);
},
child: const Text('Go to song 2'),
),
```
To learn more about navigation, see the [Navigation][] topic.
## Redirecting to a named route
To redirect to a named route, use the `namedLocation` API:
```dart
redirect: (BuildContext context, GoRouterState state) {
if (AuthState.of(context).isSignedIn) {
return context.namedLocation('signIn');
} else {
return null;
}
},
```
To learn more about redirection, see the [Redirection][] topic.
[Navigation]: https://pub.dev/documentation/go_router/latest/topics/Navigation-topic.html
[Redirection]: https://pub.dev/documentation/go_router/latest/topics/Redirection-topic.html
| packages/packages/go_router/doc/named-routes.md/0 | {
"file_path": "packages/packages/go_router/doc/named-routes.md",
"repo_id": "packages",
"token_count": 480
} | 932 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:adaptive_navigation/adaptive_navigation.dart';
import 'package:flutter/material.dart';
import 'package:go_router/go_router.dart';
/// The enum for scaffold tab.
enum ScaffoldTab {
/// The books tab.
books,
/// The authors tab.
authors,
/// The settings tab.
settings
}
/// The scaffold for the book store.
class BookstoreScaffold extends StatelessWidget {
/// Creates a [BookstoreScaffold].
const BookstoreScaffold({
required this.selectedTab,
required this.child,
super.key,
});
/// Which tab of the scaffold to display.
final ScaffoldTab selectedTab;
/// The scaffold body.
final Widget child;
@override
Widget build(BuildContext context) => Scaffold(
body: AdaptiveNavigationScaffold(
selectedIndex: selectedTab.index,
body: child,
onDestinationSelected: (int idx) {
switch (ScaffoldTab.values[idx]) {
case ScaffoldTab.books:
context.go('/books');
case ScaffoldTab.authors:
context.go('/authors');
case ScaffoldTab.settings:
context.go('/settings');
}
},
destinations: const <AdaptiveScaffoldDestination>[
AdaptiveScaffoldDestination(
title: 'Books',
icon: Icons.book,
),
AdaptiveScaffoldDestination(
title: 'Authors',
icon: Icons.person,
),
AdaptiveScaffoldDestination(
title: 'Settings',
icon: Icons.settings,
),
],
),
);
}
| packages/packages/go_router/example/lib/books/src/screens/scaffold.dart/0 | {
"file_path": "packages/packages/go_router/example/lib/books/src/screens/scaffold.dart",
"repo_id": "packages",
"token_count": 804
} | 933 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:go_router/go_router.dart';
void main() => runApp(App());
/// The main app.
class App extends StatelessWidget {
/// Creates an [App].
App({super.key});
/// The title of the app.
static const String title = 'GoRouter Example: Router neglect';
@override
Widget build(BuildContext context) => MaterialApp.router(
routerConfig: _router,
title: title,
);
final GoRouter _router = GoRouter(
// turn off history tracking in the browser for this navigation
routerNeglect: true,
routes: <GoRoute>[
GoRoute(
path: '/',
builder: (BuildContext context, GoRouterState state) =>
const Page1Screen(),
),
GoRoute(
path: '/page2',
builder: (BuildContext context, GoRouterState state) =>
const Page2Screen(),
),
],
);
}
/// The screen of the first page.
class Page1Screen extends StatelessWidget {
/// Creates a [Page1Screen].
const Page1Screen({super.key});
@override
Widget build(BuildContext context) => Scaffold(
appBar: AppBar(title: const Text(App.title)),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
onPressed: () => context.go('/page2'),
child: const Text('Go to page 2'),
),
const SizedBox(height: 8),
ElevatedButton(
// turn off history tracking in the browser for this navigation;
// note that this isn't necessary when you've set routerNeglect
// but it does illustrate the technique
onPressed: () => Router.neglect(
context,
() => context.push('/page2'),
),
child: const Text('Push page 2'),
),
],
),
),
);
}
/// The screen of the second page.
class Page2Screen extends StatelessWidget {
/// Creates a [Page2Screen].
const Page2Screen({super.key});
@override
Widget build(BuildContext context) => Scaffold(
appBar: AppBar(title: const Text(App.title)),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
onPressed: () => context.go('/'),
child: const Text('Go to home page'),
),
],
),
),
);
}
| packages/packages/go_router/example/lib/others/router_neglect.dart/0 | {
"file_path": "packages/packages/go_router/example/lib/others/router_neglect.dart",
"repo_id": "packages",
"token_count": 1214
} | 934 |
#include "../../Flutter/Flutter-Debug.xcconfig"
#include "Warnings.xcconfig"
| packages/packages/go_router/example/macos/Runner/Configs/Debug.xcconfig/0 | {
"file_path": "packages/packages/go_router/example/macos/Runner/Configs/Debug.xcconfig",
"repo_id": "packages",
"token_count": 32
} | 935 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:go_router_examples/shell_route.dart' as example;
void main() {
testWidgets('example works', (WidgetTester tester) async {
await tester.pumpWidget(example.ShellRouteExampleApp());
expect(find.text('Screen A'), findsOneWidget);
// navigate to a/details
await tester.tap(find.text('View A details'));
await tester.pumpAndSettle();
expect(find.text('Details for A'), findsOneWidget);
// navigate to ScreenB
await tester.tap(find.text('B Screen'));
await tester.pumpAndSettle();
expect(find.text('Screen B'), findsOneWidget);
// navigate to b/details
await tester.tap(find.text('View B details'));
await tester.pumpAndSettle();
expect(find.text('Details for B'), findsOneWidget);
// back to ScreenB.
await tester.tap(find.byType(BackButton));
await tester.pumpAndSettle();
// navigate to ScreenC
await tester.tap(find.text('C Screen'));
await tester.pumpAndSettle();
expect(find.text('Screen C'), findsOneWidget);
// navigate to c/details
await tester.tap(find.text('View C details'));
await tester.pumpAndSettle();
expect(find.text('Details for C'), findsOneWidget);
});
}
| packages/packages/go_router/example/test/shell_route_test.dart/0 | {
"file_path": "packages/packages/go_router/example/test/shell_route_test.dart",
"repo_id": "packages",
"token_count": 503
} | 936 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
/// Thrown when [GoRouter] is used incorrectly.
class GoError extends Error {
/// Constructs a [GoError]
GoError(this.message);
/// The error message.
final String message;
@override
String toString() => 'GoError: $message';
}
/// Thrown when [GoRouter] can not handle a user request.
class GoException implements Exception {
/// Creates an exception with message describing the reason.
GoException(this.message);
/// The reason that causes this exception.
final String message;
@override
String toString() => 'GoException: $message';
}
| packages/packages/go_router/lib/src/misc/errors.dart/0 | {
"file_path": "packages/packages/go_router/lib/src/misc/errors.dart",
"repo_id": "packages",
"token_count": 200
} | 937 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:go_router/src/pages/cupertino.dart';
import 'helpers/error_screen_helpers.dart';
void main() {
group('isCupertinoApp', () {
testWidgets('returns [true] when CupertinoApp is present',
(WidgetTester tester) async {
final GlobalKey<_DummyStatefulWidgetState> key =
GlobalKey<_DummyStatefulWidgetState>();
await tester.pumpWidget(
CupertinoApp(
home: DummyStatefulWidget(key: key),
),
);
final bool isCupertino = isCupertinoApp(key.currentContext! as Element);
expect(isCupertino, true);
});
testWidgets('returns [false] when MaterialApp is present',
(WidgetTester tester) async {
final GlobalKey<_DummyStatefulWidgetState> key =
GlobalKey<_DummyStatefulWidgetState>();
await tester.pumpWidget(
MaterialApp(
home: DummyStatefulWidget(key: key),
),
);
final bool isCupertino = isCupertinoApp(key.currentContext! as Element);
expect(isCupertino, false);
});
});
test('pageBuilderForCupertinoApp creates a [CupertinoPage] accordingly', () {
final UniqueKey key = UniqueKey();
const String name = 'name';
const String arguments = 'arguments';
const String restorationId = 'restorationId';
const DummyStatefulWidget child = DummyStatefulWidget();
final CupertinoPage<void> page = pageBuilderForCupertinoApp(
key: key,
name: name,
arguments: arguments,
restorationId: restorationId,
child: child,
);
expect(page.key, key);
expect(page.name, name);
expect(page.arguments, arguments);
expect(page.restorationId, restorationId);
expect(page.child, child);
});
group('GoRouterCupertinoErrorScreen', () {
testWidgets(
'shows "page not found" by default',
testPageNotFound(
widget: const CupertinoApp(
home: CupertinoErrorScreen(null),
),
),
);
final Exception exception = Exception('Something went wrong!');
testWidgets(
'shows the exception message when provided',
testPageShowsExceptionMessage(
exception: exception,
widget: CupertinoApp(
home: CupertinoErrorScreen(exception),
),
),
);
testWidgets(
'clicking the CupertinoButton should redirect to /',
testClickingTheButtonRedirectsToRoot(
buttonFinder: find.byType(CupertinoButton),
appRouterBuilder: cupertinoAppRouterBuilder,
widget: const CupertinoApp(
home: CupertinoErrorScreen(null),
),
),
);
});
}
class DummyStatefulWidget extends StatefulWidget {
const DummyStatefulWidget({super.key});
@override
State<DummyStatefulWidget> createState() => _DummyStatefulWidgetState();
}
class _DummyStatefulWidgetState extends State<DummyStatefulWidget> {
@override
Widget build(BuildContext context) => Container();
}
| packages/packages/go_router/test/cupertino_test.dart/0 | {
"file_path": "packages/packages/go_router/test/cupertino_test.dart",
"repo_id": "packages",
"token_count": 1238
} | 938 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/widgets.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:go_router/go_router.dart';
import 'package:go_router/src/match.dart';
import 'test_helpers.dart';
void main() {
testWidgets('RouteMatchList toString prints the fullPath',
(WidgetTester tester) async {
final List<GoRoute> routes = <GoRoute>[
GoRoute(
path: '/page-0',
builder: (BuildContext context, GoRouterState state) =>
const Placeholder()),
];
final GoRouter router =
await createRouter(routes, tester, initialLocation: '/page-0');
final RouteMatchList matches = router.routerDelegate.currentConfiguration;
expect(matches.toString(), contains('/page-0'));
});
test('RouteMatchList compares', () async {
final GoRoute route = GoRoute(
path: '/page-0',
builder: (BuildContext context, GoRouterState state) =>
const Placeholder(),
);
final Map<String, String> params1 = <String, String>{};
final List<RouteMatchBase> match1 = RouteMatchBase.match(
route: route,
uri: Uri.parse('/page-0'),
rootNavigatorKey: GlobalKey<NavigatorState>(),
pathParameters: params1,
);
final Map<String, String> params2 = <String, String>{};
final List<RouteMatchBase> match2 = RouteMatchBase.match(
route: route,
uri: Uri.parse('/page-0'),
rootNavigatorKey: GlobalKey<NavigatorState>(),
pathParameters: params2,
);
final RouteMatchList matches1 = RouteMatchList(
matches: match1,
uri: Uri.parse(''),
pathParameters: params1,
);
final RouteMatchList matches2 = RouteMatchList(
matches: match2,
uri: Uri.parse(''),
pathParameters: params2,
);
final RouteMatchList matches3 = RouteMatchList(
matches: match2,
uri: Uri.parse('/page-0'),
pathParameters: params2,
);
expect(matches1 == matches2, isTrue);
expect(matches1 == matches3, isFalse);
});
test('RouteMatchList is encoded and decoded correctly', () {
final RouteConfiguration configuration = createRouteConfiguration(
routes: <GoRoute>[
GoRoute(
path: '/a',
builder: (BuildContext context, GoRouterState state) =>
const Placeholder(),
),
GoRoute(
path: '/b',
builder: (BuildContext context, GoRouterState state) =>
const Placeholder(),
),
],
redirectLimit: 0,
navigatorKey: GlobalKey<NavigatorState>(),
topRedirect: (_, __) => null,
);
final RouteMatchListCodec codec = RouteMatchListCodec(configuration);
final RouteMatchList list1 = configuration.findMatch('/a');
final RouteMatchList list2 = configuration.findMatch('/b');
list1.push(ImperativeRouteMatch(
pageKey: const ValueKey<String>('/b-p0'),
matches: list2,
completer: Completer<Object?>()));
final Map<Object?, Object?> encoded = codec.encode(list1);
final RouteMatchList decoded = codec.decode(encoded);
expect(decoded, isNotNull);
expect(decoded, equals(list1));
});
}
| packages/packages/go_router/test/matching_test.dart/0 | {
"file_path": "packages/packages/go_router/test/matching_test.dart",
"repo_id": "packages",
"token_count": 1295
} | 939 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// Called from the custom-tests CI action.
//
// usage: dart run tool/run_tests.dart
// ignore_for_file: avoid_print
import 'dart:async';
import 'dart:io';
import 'package:io/io.dart' as io;
import 'package:path/path.dart' as p;
// This test runner simulates a consumption of go_router that checks if
// the dart fixes are applied correctly.
// This is done by copying the `test_fixes/` directory to a temp directory
// that references `go_router`, and running `dart fix --compare-to-golden`
// on the temp directory.
Future<void> main(List<String> args) async {
final Directory goRouterPackageRoot =
File.fromUri(Platform.script).parent.parent;
final Directory testTempDir = await Directory.systemTemp.createTemp();
// Cleans up the temp directory and exits with a given statusCode.
Future<Never> cleanUpAndExit(int statusCode) async {
await testTempDir.delete(recursive: true);
exit(statusCode);
}
// Copy the test_fixes folder to the temporary testFixesTargetDir.
//
// This also creates the proper pubspec.yaml in the temp directory.
await _prepareTemplate(
packageRoot: goRouterPackageRoot,
testTempDir: testTempDir,
);
// Run dart pub get in the temp directory to set it up.
final int pubGetStatusCode = await _runProcess(
'dart',
<String>[
'pub',
'get',
],
workingDirectory: testTempDir.path,
);
if (pubGetStatusCode != 0) {
await cleanUpAndExit(pubGetStatusCode);
}
// Run dart fix --compare-to-golden in the temp directory.
final int dartFixStatusCode = await _runProcess(
'dart',
<String>[
'fix',
'--compare-to-golden',
],
workingDirectory: testTempDir.path,
);
await cleanUpAndExit(dartFixStatusCode);
}
Future<void> _prepareTemplate({
required Directory packageRoot,
required Directory testTempDir,
}) async {
// The src test_fixes directory.
final Directory testFixesSrcDir =
Directory(p.join(packageRoot.path, 'test_fixes'));
// Copy from src `test_fixes/` to the temp directory.
await io.copyPath(testFixesSrcDir.path, testTempDir.path);
// The pubspec.yaml file to create.
final File targetPubspecFile = File(p.join(testTempDir.path, 'pubspec.yaml'));
final String targetYaml = '''
name: test_fixes
publish_to: "none"
version: 1.0.0
environment:
sdk: ">=2.18.0 <4.0.0"
flutter: ">=3.3.0"
dependencies:
flutter:
sdk: flutter
go_router:
path: ${packageRoot.path}
''';
await targetPubspecFile.writeAsString(targetYaml);
}
Future<Process> _streamOutput(Future<Process> processFuture) async {
final Process process = await processFuture;
unawaited(stdout.addStream(process.stdout));
unawaited(stderr.addStream(process.stderr));
return process;
}
Future<int> _runProcess(
String command,
List<String> arguments, {
String? workingDirectory,
}) async {
final Process process = await _streamOutput(Process.start(
command,
arguments,
workingDirectory: workingDirectory,
));
return process.exitCode;
}
| packages/packages/go_router/tool/run_tests.dart/0 | {
"file_path": "packages/packages/go_router/tool/run_tests.dart",
"repo_id": "packages",
"token_count": 1071
} | 940 |
// GENERATED CODE - DO NOT MODIFY BY HAND
// ignore_for_file: always_specify_types, public_member_api_docs
part of 'main.dart';
// **************************************************************************
// GoRouterGenerator
// **************************************************************************
List<RouteBase> get $appRoutes => [
$homeRoute,
$loginRoute,
];
RouteBase get $homeRoute => GoRouteData.$route(
path: '/',
factory: $HomeRouteExtension._fromState,
routes: [
GoRouteData.$route(
path: 'family/:fid',
factory: $FamilyRouteExtension._fromState,
routes: [
GoRouteData.$route(
path: 'person/:pid',
factory: $PersonRouteExtension._fromState,
routes: [
GoRouteData.$route(
path: 'details/:details',
factory: $PersonDetailsRouteExtension._fromState,
),
],
),
],
),
GoRouteData.$route(
path: 'family-count/:count',
factory: $FamilyCountRouteExtension._fromState,
),
],
);
extension $HomeRouteExtension on HomeRoute {
static HomeRoute _fromState(GoRouterState state) => const HomeRoute();
String get location => GoRouteData.$location(
'/',
);
void go(BuildContext context) => context.go(location);
Future<T?> push<T>(BuildContext context) => context.push<T>(location);
void pushReplacement(BuildContext context) =>
context.pushReplacement(location);
void replace(BuildContext context) => context.replace(location);
}
extension $FamilyRouteExtension on FamilyRoute {
static FamilyRoute _fromState(GoRouterState state) => FamilyRoute(
state.pathParameters['fid']!,
);
String get location => GoRouteData.$location(
'/family/${Uri.encodeComponent(fid)}',
);
void go(BuildContext context) => context.go(location);
Future<T?> push<T>(BuildContext context) => context.push<T>(location);
void pushReplacement(BuildContext context) =>
context.pushReplacement(location);
void replace(BuildContext context) => context.replace(location);
}
extension $PersonRouteExtension on PersonRoute {
static PersonRoute _fromState(GoRouterState state) => PersonRoute(
state.pathParameters['fid']!,
int.parse(state.pathParameters['pid']!),
);
String get location => GoRouteData.$location(
'/family/${Uri.encodeComponent(fid)}/person/${Uri.encodeComponent(pid.toString())}',
);
void go(BuildContext context) => context.go(location);
Future<T?> push<T>(BuildContext context) => context.push<T>(location);
void pushReplacement(BuildContext context) =>
context.pushReplacement(location);
void replace(BuildContext context) => context.replace(location);
}
extension $PersonDetailsRouteExtension on PersonDetailsRoute {
static PersonDetailsRoute _fromState(GoRouterState state) =>
PersonDetailsRoute(
state.pathParameters['fid']!,
int.parse(state.pathParameters['pid']!),
_$PersonDetailsEnumMap._$fromName(state.pathParameters['details']!),
$extra: state.extra as int?,
);
String get location => GoRouteData.$location(
'/family/${Uri.encodeComponent(fid)}/person/${Uri.encodeComponent(pid.toString())}/details/${Uri.encodeComponent(_$PersonDetailsEnumMap[details]!)}',
);
void go(BuildContext context) => context.go(location, extra: $extra);
Future<T?> push<T>(BuildContext context) =>
context.push<T>(location, extra: $extra);
void pushReplacement(BuildContext context) =>
context.pushReplacement(location, extra: $extra);
void replace(BuildContext context) =>
context.replace(location, extra: $extra);
}
const _$PersonDetailsEnumMap = {
PersonDetails.hobbies: 'hobbies',
PersonDetails.favoriteFood: 'favorite-food',
PersonDetails.favoriteSport: 'favorite-sport',
};
extension $FamilyCountRouteExtension on FamilyCountRoute {
static FamilyCountRoute _fromState(GoRouterState state) => FamilyCountRoute(
int.parse(state.pathParameters['count']!),
);
String get location => GoRouteData.$location(
'/family-count/${Uri.encodeComponent(count.toString())}',
);
void go(BuildContext context) => context.go(location);
Future<T?> push<T>(BuildContext context) => context.push<T>(location);
void pushReplacement(BuildContext context) =>
context.pushReplacement(location);
void replace(BuildContext context) => context.replace(location);
}
extension<T extends Enum> on Map<T, String> {
T _$fromName(String value) =>
entries.singleWhere((element) => element.value == value).key;
}
RouteBase get $loginRoute => GoRouteData.$route(
path: '/login',
factory: $LoginRouteExtension._fromState,
);
extension $LoginRouteExtension on LoginRoute {
static LoginRoute _fromState(GoRouterState state) => LoginRoute(
fromPage: state.uri.queryParameters['from-page'],
);
String get location => GoRouteData.$location(
'/login',
queryParams: {
if (fromPage != null) 'from-page': fromPage,
},
);
void go(BuildContext context) => context.go(location);
Future<T?> push<T>(BuildContext context) => context.push<T>(location);
void pushReplacement(BuildContext context) =>
context.pushReplacement(location);
void replace(BuildContext context) => context.replace(location);
}
| packages/packages/go_router_builder/example/lib/main.g.dart/0 | {
"file_path": "packages/packages/go_router_builder/example/lib/main.g.dart",
"repo_id": "packages",
"token_count": 1975
} | 941 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:build_verify/build_verify.dart';
import 'package:flutter_test/flutter_test.dart';
void main() {
test(
'ensure_build',
() => expectBuildClean(
packageRelativeDirectory: 'packages/go_router_builder/example',
gitDiffPathArguments: <String>[':!pubspec.yaml'],
),
timeout: const Timeout.factor(3),
);
}
| packages/packages/go_router_builder/example/test/ensure_build_test.dart/0 | {
"file_path": "packages/packages/go_router_builder/example/test/ensure_build_test.dart",
"repo_id": "packages",
"token_count": 179
} | 942 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:go_router/go_router.dart';
@TypedGoRoute<DefaultValueRoute>(path: '/default-value-route')
class DefaultValueRoute extends GoRouteData {
DefaultValueRoute({this.param = 0});
final int param;
}
| packages/packages/go_router_builder/test_inputs/default_value.dart/0 | {
"file_path": "packages/packages/go_router_builder/test_inputs/default_value.dart",
"repo_id": "packages",
"token_count": 111
} | 943 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:go_router/go_router.dart';
@TypedGoRoute<NullableDefaultValueRoute>(path: '/nullable-default-value-route')
class NullableDefaultValueRoute extends GoRouteData {
NullableDefaultValueRoute({this.param = 0});
final int? param;
}
| packages/packages/go_router_builder/test_inputs/nullable_default_value.dart/0 | {
"file_path": "packages/packages/go_router_builder/test_inputs/nullable_default_value.dart",
"repo_id": "packages",
"token_count": 121
} | 944 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// the following ignore is needed for downgraded analyzer (casts to JSObject).
// ignore_for_file: unnecessary_cast
import 'dart:async';
import 'dart:js_interop';
import 'package:flutter_test/flutter_test.dart';
import 'package:google_identity_services_web/oauth2.dart';
import 'package:integration_test/integration_test.dart';
import 'utils.dart' as utils;
void main() async {
IntegrationTestWidgetsFlutterBinding.ensureInitialized();
setUpAll(() async {
// Load web/mock-gis.js in the page
await utils.installGisMock();
});
group('Config objects pass values from Dart to JS - ', () {
testWidgets('TokenClientConfig', (_) async {
final TokenClientConfig config = TokenClientConfig(
client_id: 'testing_1-2-3',
callback: (_) {},
scope: <String>['one', 'two', 'three'],
include_granted_scopes: true,
prompt: 'some-prompt',
enable_granular_consent: true,
login_hint: '[email protected]',
hd: 'hd_value',
state: 'some-state',
error_callback: (_) {},
);
final utils.ExpectConfigValueFn expectConfigValue =
utils.createExpectConfigValue(config as JSObject);
expectConfigValue('client_id', 'testing_1-2-3');
expectConfigValue('callback', utils.isAJs('function'));
expectConfigValue('scope', 'one two three');
expectConfigValue('include_granted_scopes', isTrue);
expectConfigValue('prompt', 'some-prompt');
expectConfigValue('enable_granular_consent', isTrue);
expectConfigValue('login_hint', '[email protected]');
expectConfigValue('hd', 'hd_value');
expectConfigValue('state', 'some-state');
expectConfigValue('error_callback', utils.isAJs('function'));
});
testWidgets('OverridableTokenClientConfig', (_) async {
final OverridableTokenClientConfig config = OverridableTokenClientConfig(
scope: <String>['one', 'two', 'three'],
include_granted_scopes: true,
prompt: 'some-prompt',
enable_granular_consent: true,
login_hint: '[email protected]',
state: 'some-state',
);
final utils.ExpectConfigValueFn expectConfigValue =
utils.createExpectConfigValue(config as JSObject);
expectConfigValue('scope', 'one two three');
expectConfigValue('include_granted_scopes', isTrue);
expectConfigValue('prompt', 'some-prompt');
expectConfigValue('enable_granular_consent', isTrue);
expectConfigValue('login_hint', '[email protected]');
expectConfigValue('state', 'some-state');
});
testWidgets('CodeClientConfig', (_) async {
final CodeClientConfig config = CodeClientConfig(
client_id: 'testing_1-2-3',
scope: <String>['one', 'two', 'three'],
include_granted_scopes: true,
redirect_uri: Uri.parse('https://www.example.com/login'),
callback: (_) {},
state: 'some-state',
enable_granular_consent: true,
login_hint: '[email protected]',
hd: 'hd_value',
ux_mode: UxMode.popup,
select_account: true,
error_callback: (_) {},
);
final utils.ExpectConfigValueFn expectConfigValue =
utils.createExpectConfigValue(config as JSObject);
expectConfigValue('scope', 'one two three');
expectConfigValue('include_granted_scopes', isTrue);
expectConfigValue('redirect_uri', 'https://www.example.com/login');
expectConfigValue('callback', utils.isAJs('function'));
expectConfigValue('state', 'some-state');
expectConfigValue('enable_granular_consent', isTrue);
expectConfigValue('login_hint', '[email protected]');
expectConfigValue('hd', 'hd_value');
expectConfigValue('ux_mode', 'popup');
expectConfigValue('select_account', isTrue);
expectConfigValue('error_callback', utils.isAJs('function'));
});
});
group('initTokenClient', () {
testWidgets('returns a tokenClient', (_) async {
final TokenClient client = oauth2.initTokenClient(TokenClientConfig(
client_id: 'for-tests',
callback: (_) {},
scope: <String>['some_scope', 'for_tests', 'not_real'],
));
expect(client, isNotNull);
});
});
group('requestAccessToken', () {
testWidgets('passes through configuration', (_) async {
final StreamController<TokenResponse> controller =
StreamController<TokenResponse>();
final List<String> scopes = <String>['some_scope', 'another', 'more'];
final TokenClient client = oauth2.initTokenClient(TokenClientConfig(
client_id: 'for-tests',
callback: controller.add,
scope: scopes,
));
utils.setMockTokenResponse(client, 'some-non-null-auth-token-value');
client.requestAccessToken();
final TokenResponse response = await controller.stream.first;
expect(response, isNotNull);
expect(response.error, isNull);
expect(response.scope, scopes);
});
testWidgets('configuration can be overridden', (_) async {
final StreamController<TokenResponse> controller =
StreamController<TokenResponse>();
final List<String> scopes = <String>['some_scope', 'another', 'more'];
final TokenClient client = oauth2.initTokenClient(TokenClientConfig(
client_id: 'for-tests',
callback: controller.add,
scope: <String>['blank'],
));
utils.setMockTokenResponse(client, 'some-non-null-auth-token-value');
client.requestAccessToken(OverridableTokenClientConfig(
scope: scopes,
));
final TokenResponse response = await controller.stream.first;
expect(response, isNotNull);
expect(response.error, isNull);
expect(response.scope, scopes);
});
});
group('hasGranted...Scopes', () {
// mock-gis.js returns false for scopes that start with "not-granted-".
const String notGranted = 'not-granted-scope';
testWidgets('all scopes granted', (_) async {
final List<String> scopes = <String>['some_scope', 'another', 'more'];
final TokenResponse response = await utils.fakeAuthZWithScopes(scopes);
final bool all = oauth2.hasGrantedAllScopes(response, scopes);
final bool any = oauth2.hasGrantedAnyScopes(response, scopes);
expect(all, isTrue);
expect(any, isTrue);
});
testWidgets('some scopes granted', (_) async {
final List<String> scopes = <String>['some_scope', notGranted, 'more'];
final TokenResponse response = await utils.fakeAuthZWithScopes(scopes);
final bool all = oauth2.hasGrantedAllScopes(response, scopes);
final bool any = oauth2.hasGrantedAnyScopes(response, scopes);
expect(all, isFalse, reason: 'Scope: $notGranted should not be granted!');
expect(any, isTrue);
});
testWidgets('no scopes granted', (_) async {
final List<String> scopes = <String>[notGranted, '$notGranted-2'];
final TokenResponse response = await utils.fakeAuthZWithScopes(scopes);
final bool all = oauth2.hasGrantedAllScopes(response, scopes);
final bool any = oauth2.hasGrantedAnyScopes(response, scopes);
expect(all, isFalse);
expect(any, isFalse, reason: 'No scopes were granted.');
});
});
}
| packages/packages/google_identity_services_web/example/integration_test/js_interop_oauth_test.dart/0 | {
"file_path": "packages/packages/google_identity_services_web/example/integration_test/js_interop_oauth_test.dart",
"repo_id": "packages",
"token_count": 2858
} | 945 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'package:web/web.dart' as web;
/// Injects a `<meta>` tag with the provided [attributes] into the [web.document].
void injectMetaTag(Map<String, String> attributes) {
final web.HTMLMetaElement meta =
web.document.createElement('meta') as web.HTMLMetaElement;
for (final MapEntry<String, String> attribute in attributes.entries) {
meta.setAttribute(attribute.key, attribute.value);
}
web.document.head!.appendChild(meta);
}
| packages/packages/google_identity_services_web/test/tools.dart/0 | {
"file_path": "packages/packages/google_identity_services_web/test/tools.dart",
"repo_id": "packages",
"token_count": 186
} | 946 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
import 'dart:async';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:google_maps_flutter/google_maps_flutter.dart';
import 'package:google_maps_flutter_platform_interface/google_maps_flutter_platform_interface.dart';
import 'package:integration_test/integration_test.dart';
import 'shared.dart';
/// Integration Tests that use the [GoogleMapsInspectorPlatform].
void main() {
IntegrationTestWidgetsFlutterBinding.ensureInitialized();
runTests();
}
void runTests() {
GoogleMapsFlutterPlatform.instance.enableDebugInspection();
final GoogleMapsInspectorPlatform inspector =
GoogleMapsInspectorPlatform.instance!;
testWidgets('testCompassToggle', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
compassEnabled: false,
onMapCreated: (GoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
);
final int mapId = await mapIdCompleter.future;
bool compassEnabled = await inspector.isCompassEnabled(mapId: mapId);
expect(compassEnabled, false);
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
onMapCreated: (GoogleMapController controller) {
fail('OnMapCreated should get called only once.');
},
),
);
compassEnabled = await inspector.isCompassEnabled(mapId: mapId);
expect(compassEnabled, !kIsWeb);
});
testWidgets('testMapToolbarToggle', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
mapToolbarEnabled: false,
onMapCreated: (GoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
);
final int mapId = await mapIdCompleter.future;
bool mapToolbarEnabled = await inspector.isMapToolbarEnabled(mapId: mapId);
expect(mapToolbarEnabled, false);
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
onMapCreated: (GoogleMapController controller) {
fail('OnMapCreated should get called only once.');
},
),
);
mapToolbarEnabled = await inspector.isMapToolbarEnabled(mapId: mapId);
expect(mapToolbarEnabled, isAndroid);
});
testWidgets('updateMinMaxZoomLevels', (WidgetTester tester) async {
// The behaviors of setting min max zoom level on iOS and Android are different.
// On iOS, when we get the min or max zoom level after setting the preference, the
// min and max will be exactly the same as the value we set; on Android however,
// the values we get do not equal to the value we set.
//
// Also, when we call zoomTo to set the zoom, on Android, it usually
// honors the preferences that we set and the zoom cannot pass beyond the boundary.
// On iOS, on the other hand, zoomTo seems to override the preferences.
//
// Thus we test iOS and Android a little differently here.
final Key key = GlobalKey();
final Completer<GoogleMapController> controllerCompleter =
Completer<GoogleMapController>();
const MinMaxZoomPreference initialZoomLevel = MinMaxZoomPreference(4, 8);
const MinMaxZoomPreference finalZoomLevel = MinMaxZoomPreference(6, 10);
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
minMaxZoomPreference: initialZoomLevel,
onMapCreated: (GoogleMapController c) async {
controllerCompleter.complete(c);
},
),
);
final GoogleMapController controller = await controllerCompleter.future;
if (isIOS) {
final MinMaxZoomPreference zoomLevel =
await inspector.getMinMaxZoomLevels(mapId: controller.mapId);
expect(zoomLevel, equals(initialZoomLevel));
} else if (isAndroid) {
await controller.moveCamera(CameraUpdate.zoomTo(15));
await tester.pumpAndSettle();
double? zoomLevel = await controller.getZoomLevel();
expect(zoomLevel, equals(initialZoomLevel.maxZoom));
await controller.moveCamera(CameraUpdate.zoomTo(1));
await tester.pumpAndSettle();
zoomLevel = await controller.getZoomLevel();
expect(zoomLevel, equals(initialZoomLevel.minZoom));
}
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
minMaxZoomPreference: finalZoomLevel,
onMapCreated: (GoogleMapController controller) {
fail('OnMapCreated should get called only once.');
},
),
);
if (isIOS) {
final MinMaxZoomPreference zoomLevel =
await inspector.getMinMaxZoomLevels(mapId: controller.mapId);
expect(zoomLevel, equals(finalZoomLevel));
} else {
await controller.moveCamera(CameraUpdate.zoomTo(15));
await tester.pumpAndSettle();
double? zoomLevel = await controller.getZoomLevel();
expect(zoomLevel, equals(finalZoomLevel.maxZoom));
await controller.moveCamera(CameraUpdate.zoomTo(1));
await tester.pumpAndSettle();
zoomLevel = await controller.getZoomLevel();
expect(zoomLevel, equals(finalZoomLevel.minZoom));
}
});
testWidgets('testZoomGesturesEnabled', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
zoomGesturesEnabled: false,
onMapCreated: (GoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
);
final int mapId = await mapIdCompleter.future;
bool zoomGesturesEnabled =
await inspector.areZoomGesturesEnabled(mapId: mapId);
expect(zoomGesturesEnabled, false);
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
onMapCreated: (GoogleMapController controller) {
fail('OnMapCreated should get called only once.');
},
),
);
zoomGesturesEnabled = await inspector.areZoomGesturesEnabled(mapId: mapId);
expect(zoomGesturesEnabled, true);
});
testWidgets('testZoomControlsEnabled', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
onMapCreated: (GoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
);
final int mapId = await mapIdCompleter.future;
bool zoomControlsEnabled =
await inspector.areZoomControlsEnabled(mapId: mapId);
expect(zoomControlsEnabled, !isIOS);
/// Zoom Controls functionality is not available on iOS at the moment.
if (!isIOS) {
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
zoomControlsEnabled: false,
onMapCreated: (GoogleMapController controller) {
fail('OnMapCreated should get called only once.');
},
),
);
zoomControlsEnabled =
await inspector.areZoomControlsEnabled(mapId: mapId);
expect(zoomControlsEnabled, false);
}
});
testWidgets('testLiteModeEnabled', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
onMapCreated: (GoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
);
final int mapId = await mapIdCompleter.future;
bool liteModeEnabled = await inspector.isLiteModeEnabled(mapId: mapId);
expect(liteModeEnabled, false);
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
liteModeEnabled: true,
onMapCreated: (GoogleMapController controller) {
fail('OnMapCreated should get called only once.');
},
),
);
liteModeEnabled = await inspector.isLiteModeEnabled(mapId: mapId);
expect(liteModeEnabled, true);
}, skip: !isAndroid);
testWidgets('testRotateGesturesEnabled', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
rotateGesturesEnabled: false,
onMapCreated: (GoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
);
final int mapId = await mapIdCompleter.future;
bool rotateGesturesEnabled =
await inspector.areRotateGesturesEnabled(mapId: mapId);
expect(rotateGesturesEnabled, false);
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
onMapCreated: (GoogleMapController controller) {
fail('OnMapCreated should get called only once.');
},
),
);
rotateGesturesEnabled =
await inspector.areRotateGesturesEnabled(mapId: mapId);
expect(rotateGesturesEnabled, !isWeb);
});
testWidgets('testTiltGesturesEnabled', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
tiltGesturesEnabled: false,
onMapCreated: (GoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
);
final int mapId = await mapIdCompleter.future;
bool tiltGesturesEnabled =
await inspector.areTiltGesturesEnabled(mapId: mapId);
expect(tiltGesturesEnabled, false);
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
onMapCreated: (GoogleMapController controller) {
fail('OnMapCreated should get called only once.');
},
),
);
tiltGesturesEnabled = await inspector.areTiltGesturesEnabled(mapId: mapId);
expect(tiltGesturesEnabled, !isWeb);
});
testWidgets('testScrollGesturesEnabled', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
scrollGesturesEnabled: false,
onMapCreated: (GoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
);
final int mapId = await mapIdCompleter.future;
bool scrollGesturesEnabled =
await inspector.areScrollGesturesEnabled(mapId: mapId);
expect(scrollGesturesEnabled, false);
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
onMapCreated: (GoogleMapController controller) {
fail('OnMapCreated should get called only once.');
},
),
);
scrollGesturesEnabled =
await inspector.areScrollGesturesEnabled(mapId: mapId);
expect(scrollGesturesEnabled, true);
});
testWidgets('testTraffic', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
trafficEnabled: true,
onMapCreated: (GoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
);
final int mapId = await mapIdCompleter.future;
bool isTrafficEnabled = await inspector.isTrafficEnabled(mapId: mapId);
expect(isTrafficEnabled, true);
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
onMapCreated: (GoogleMapController controller) {
fail('OnMapCreated should get called only once.');
},
),
);
isTrafficEnabled = await inspector.isTrafficEnabled(mapId: mapId);
expect(isTrafficEnabled, false);
});
testWidgets('testBuildings', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
onMapCreated: (GoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
);
final int mapId = await mapIdCompleter.future;
final bool isBuildingsEnabled =
await inspector.areBuildingsEnabled(mapId: mapId);
expect(isBuildingsEnabled, !isWeb);
});
// Location button tests are skipped in Android because we don't have location permission to test.
// Location button tests are skipped in Web because the functionality is not implemented.
group('MyLocationButton', () {
testWidgets('testMyLocationButtonToggle', (WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
onMapCreated: (GoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
);
final int mapId = await mapIdCompleter.future;
bool myLocationButtonEnabled =
await inspector.isMyLocationButtonEnabled(mapId: mapId);
expect(myLocationButtonEnabled, true);
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
myLocationButtonEnabled: false,
onMapCreated: (GoogleMapController controller) {
fail('OnMapCreated should get called only once.');
},
),
);
myLocationButtonEnabled =
await inspector.isMyLocationButtonEnabled(mapId: mapId);
expect(myLocationButtonEnabled, false);
});
testWidgets('testMyLocationButton initial value false',
(WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
myLocationButtonEnabled: false,
onMapCreated: (GoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
);
final int mapId = await mapIdCompleter.future;
final bool myLocationButtonEnabled =
await inspector.isMyLocationButtonEnabled(mapId: mapId);
expect(myLocationButtonEnabled, false);
});
testWidgets('testMyLocationButton initial value true',
(WidgetTester tester) async {
final Key key = GlobalKey();
final Completer<int> mapIdCompleter = Completer<int>();
await pumpMap(
tester,
GoogleMap(
key: key,
initialCameraPosition: kInitialCameraPosition,
onMapCreated: (GoogleMapController controller) {
mapIdCompleter.complete(controller.mapId);
},
),
);
final int mapId = await mapIdCompleter.future;
final bool myLocationButtonEnabled =
await inspector.isMyLocationButtonEnabled(mapId: mapId);
expect(myLocationButtonEnabled, true);
});
}, skip: !isIOS);
}
| packages/packages/google_maps_flutter/google_maps_flutter/example/integration_test/src/maps_inspector.dart/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter/example/integration_test/src/maps_inspector.dart",
"repo_id": "packages",
"token_count": 6535
} | 947 |
## 2.7.0
* Adds support for `MapConfiguration.style`.
* Adds support for `getStyleError`.
* Updates minimum supported SDK version to Flutter 3.13/Dart 3.1.
* Updates compileSdk version to 34.
## 2.6.2
* Updates minimum required plugin_platform_interface version to 2.1.7.
## 2.6.1
* Fixes new lint warnings.
## 2.6.0
* Fixes missing updates in TLHC mode.
* Switched default display mode to TLHC mode.
* Updates minimum supported SDK version to Flutter 3.10/Dart 3.0.
## 2.5.3
* Updates `com.google.android.gms:play-services-maps` to 18.2.0.
## 2.5.2
* Updates annotations lib to 1.7.0.
## 2.5.1
* Adds pub topics to package metadata.
## 2.5.0
* Adds implementation for `cloudMapId` parameter to support cloud-based map styling.
* Updates minimum supported SDK version to Flutter 3.7/Dart 2.19.
## 2.4.16
* Removes old empty override methods.
* Fixes unawaited_futures violations.
## 2.4.15
* Removes obsolete null checks on non-nullable values.
* Updates minimum supported SDK version to Flutter 3.3/Dart 2.18.
## 2.4.14
* Updates gradle, AGP and fixes some lint errors.
## 2.4.13
* Fixes compatibility with AGP versions older than 4.2.
## 2.4.12
* Fixes Java warnings.
## 2.4.11
* Adds a namespace for compatibility with AGP 8.0.
## 2.4.10
* Bump RoboElectric dependency to 4.4.1 to support AndroidX.
## 2.4.9
* Clarifies explanation of endorsement in README.
* Aligns Dart and Flutter SDK constraints.
## 2.4.8
* Fixes compilation warnings.
## 2.4.7
* Updates annotation dependency.
* Updates compileSdkVersion to 33.
## 2.4.6
* Updates links for the merge of flutter/plugins into flutter/packages.
## 2.4.5
* Fixes Initial padding not working when map has not been created yet.
## 2.4.4
* Fixes Points losing precision when converting to LatLng.
* Updates minimum Flutter version to 3.0.
## 2.4.3
* Updates code for stricter lint checks.
## 2.4.2
* Updates code for stricter lint checks.
## 2.4.1
* Update `androidx.test.espresso:espresso-core` to 3.5.1.
## 2.4.0
* Adds the ability to request a specific map renderer.
* Updates code for new analysis options.
## 2.3.3
* Update android gradle plugin to 7.3.1.
## 2.3.2
* Update `com.google.android.gms:play-services-maps` to 18.1.0.
## 2.3.1
* Updates imports for `prefer_relative_imports`.
## 2.3.0
* Switches the default for `useAndroidViewSurface` to true, and adds
information about the current mode behaviors to the README.
* Updates minimum Flutter version to 2.10.
## 2.2.0
* Updates `useAndroidViewSurface` to require Hybrid Composition, making the
selection work again in Flutter 3.0+. Earlier versions of Flutter are
no longer supported.
* Fixes violations of new analysis option use_named_constants.
* Fixes avoid_redundant_argument_values lint warnings and minor typos.
## 2.1.10
* Splits Android implementation out of `google_maps_flutter` as a federated
implementation.
| packages/packages/google_maps_flutter/google_maps_flutter_android/CHANGELOG.md/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_android/CHANGELOG.md",
"repo_id": "packages",
"token_count": 972
} | 948 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.googlemaps;
import androidx.annotation.Nullable;
import com.google.android.gms.maps.model.LatLngBounds;
import java.util.List;
import java.util.Map;
/** Receiver of GoogleMap configuration options. */
interface GoogleMapOptionsSink {
void setCameraTargetBounds(LatLngBounds bounds);
void setCompassEnabled(boolean compassEnabled);
void setMapToolbarEnabled(boolean setMapToolbarEnabled);
void setMapType(int mapType);
void setMinMaxZoomPreference(Float min, Float max);
void setPadding(float top, float left, float bottom, float right);
void setRotateGesturesEnabled(boolean rotateGesturesEnabled);
void setScrollGesturesEnabled(boolean scrollGesturesEnabled);
void setTiltGesturesEnabled(boolean tiltGesturesEnabled);
void setTrackCameraPosition(boolean trackCameraPosition);
void setZoomGesturesEnabled(boolean zoomGesturesEnabled);
void setLiteModeEnabled(boolean liteModeEnabled);
void setMyLocationEnabled(boolean myLocationEnabled);
void setZoomControlsEnabled(boolean zoomControlsEnabled);
void setMyLocationButtonEnabled(boolean myLocationButtonEnabled);
void setIndoorEnabled(boolean indoorEnabled);
void setTrafficEnabled(boolean trafficEnabled);
void setBuildingsEnabled(boolean buildingsEnabled);
void setInitialMarkers(Object initialMarkers);
void setInitialPolygons(Object initialPolygons);
void setInitialPolylines(Object initialPolylines);
void setInitialCircles(Object initialCircles);
void setInitialTileOverlays(List<Map<String, ?>> initialTileOverlays);
void setMapStyle(@Nullable String style);
}
| packages/packages/google_maps_flutter/google_maps_flutter_android/android/src/main/java/io/flutter/plugins/googlemaps/GoogleMapOptionsSink.java/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_android/android/src/main/java/io/flutter/plugins/googlemaps/GoogleMapOptionsSink.java",
"repo_id": "packages",
"token_count": 501
} | 949 |
// Copyright 2013 The Flutter Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
package io.flutter.plugins.googlemaps;
import com.google.android.gms.maps.model.TileOverlay;
import com.google.android.gms.maps.model.TileProvider;
import java.util.HashMap;
import java.util.Map;
class TileOverlayController implements TileOverlaySink {
private final TileOverlay tileOverlay;
TileOverlayController(TileOverlay tileOverlay) {
this.tileOverlay = tileOverlay;
}
void remove() {
tileOverlay.remove();
}
void clearTileCache() {
tileOverlay.clearTileCache();
}
Map<String, Object> getTileOverlayInfo() {
Map<String, Object> tileOverlayInfo = new HashMap<>();
tileOverlayInfo.put("fadeIn", tileOverlay.getFadeIn());
tileOverlayInfo.put("transparency", tileOverlay.getTransparency());
tileOverlayInfo.put("id", tileOverlay.getId());
tileOverlayInfo.put("zIndex", tileOverlay.getZIndex());
tileOverlayInfo.put("visible", tileOverlay.isVisible());
return tileOverlayInfo;
}
@Override
public void setFadeIn(boolean fadeIn) {
tileOverlay.setFadeIn(fadeIn);
}
@Override
public void setTransparency(float transparency) {
tileOverlay.setTransparency(transparency);
}
@Override
public void setZIndex(float zIndex) {
tileOverlay.setZIndex(zIndex);
}
@Override
public void setVisible(boolean visible) {
tileOverlay.setVisible(visible);
}
@Override
public void setTileProvider(TileProvider tileProvider) {
// You can not change tile provider after creation
}
}
| packages/packages/google_maps_flutter/google_maps_flutter_android/android/src/main/java/io/flutter/plugins/googlemaps/TileOverlayController.java/0 | {
"file_path": "packages/packages/google_maps_flutter/google_maps_flutter_android/android/src/main/java/io/flutter/plugins/googlemaps/TileOverlayController.java",
"repo_id": "packages",
"token_count": 544
} | 950 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.