html_url
stringlengths 48
51
| title
stringlengths 5
268
| comments
stringlengths 63
51.8k
| body
stringlengths 0
36.2k
⌀ | comment_length
int64 16
1.52k
| text
stringlengths 164
54.1k
| embeddings
list |
---|---|---|---|---|---|---|
https://github.com/huggingface/datasets/issues/2005 | Setting to torch format not working with torchvision and MNIST | # Convert raw tensors to torch format
Strangely, converting to torch tensors works perfectly on `raw_dataset`:
```python
raw_dataset.set_format('torch',columns=['image','label'])
```
Types:
```
Image:
<class 'torch.Tensor'> 60000
<class 'torch.Tensor'> 28
<class 'torch.Tensor'> 28
<class 'torch.Tensor'>
Label:
<class 'torch.Tensor'> 60000
<class 'torch.Tensor'>
```
Using this for transforms:
```python
def prepare_features(examples):
images = []
labels = []
for example_idx, example in enumerate(examples["image"]):
if transform is not None:
images.append(transform(
examples["image"][example_idx].numpy()
))
else:
images.append(examples["image"][example_idx].numpy())
labels.append(examples["label"][example_idx])
output = {"label":labels, "image":images}
return output
```
Inside `prepare_train_features`:
```
Image:
<class 'list'> 10000
<class 'torch.Tensor'> 1
<class 'torch.Tensor'> 28
<class 'torch.Tensor'> 28
<class 'torch.Tensor'>
Label:
<class 'list'> 10000
<class 'torch.Tensor'>
```
After `map`:
```
Image:
<class 'list'> 60000
<class 'list'> 1
<class 'list'> 28
<class 'torch.Tensor'> 28
<class 'torch.Tensor'>
Label:
<class 'torch.Tensor'> 60000
<class 'torch.Tensor'>
```
DataLoader batch:
```
Image:
<class 'list'> 1
<class 'list'> 28
<class 'torch.Tensor'> 2
<class 'torch.Tensor'> 28
<class 'torch.Tensor'>
Label:
<class 'torch.Tensor'> 2
<class 'torch.Tensor'>
```
---
## Using `torch` format:
```
Image:
<class 'list'> 60000
<class 'list'> 1
<class 'list'> 28
<class 'torch.Tensor'> 28
<class 'torch.Tensor'>
Label:
<class 'torch.Tensor'> 60000
<class 'torch.Tensor'>
```
DataLoader batches:
```
Image:
<class 'list'> 1
<class 'list'> 28
<class 'torch.Tensor'> 2
<class 'torch.Tensor'> 28
<class 'torch.Tensor'>
Label:
<class 'torch.Tensor'> 2
<class 'torch.Tensor'>
```
---
## Using the features - `Array3D`:
```
Image:
<class 'list'> 10000
<class 'torch.Tensor'> 1
<class 'torch.Tensor'> 28
<class 'torch.Tensor'> 28
<class 'torch.Tensor'>
Label:
<class 'list'> 10000
<class 'torch.Tensor'>
```
After `map`:
```
Image:
<class 'torch.Tensor'> 60000
<class 'torch.Tensor'> 1
<class 'torch.Tensor'> 28
<class 'torch.Tensor'> 28
<class 'torch.Tensor'>
Label:
<class 'torch.Tensor'> 60000
<class 'torch.Tensor'>
```
After DataLoader `batch`:
```
Image:
<class 'torch.Tensor'> 2
<class 'torch.Tensor'> 1
<class 'torch.Tensor'> 28
<class 'torch.Tensor'> 28
<class 'torch.Tensor'>
Label:
<class 'torch.Tensor'> 2
<class 'torch.Tensor'>
```
The last one works perfectly.
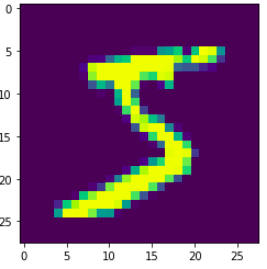
I wonder why this worked, and others didn't.
| Hi
I am trying to use `torchvision.transforms` to handle the transformation of the image data in the `mnist` dataset. Assume I have a `transform` variable which contains the `torchvision.transforms` object.
A snippet of what I am trying to do:
```python
def prepare_features(examples):
images = []
labels = []
for example_idx, example in enumerate(examples["image"]):
if transform is not None:
images.append(transform(
np.array(examples["image"][example_idx], dtype=np.uint8)
))
else:
images.append(torch.tensor(np.array(examples["image"][example_idx], dtype=np.uint8)))
labels.append(torch.tensor(examples["label"][example_idx]))
output = {"label":labels, "image":images}
return output
raw_dataset = load_dataset('mnist')
train_dataset = raw_dataset.map(prepare_features, batched=True, batch_size=10000)
train_dataset.set_format("torch",columns=["image","label"])
```
After this, I check the type of the following:
```python
print(type(train_dataset["train"]["label"]))
print(type(train_dataset["train"]["image"][0]))
```
This leads to the following output:
```python
<class 'torch.Tensor'>
<class 'list'>
```
I use `torch.utils.DataLoader` for batches, the type of `batch["train"]["image"]` is also `<class 'list'>`.
I don't understand why only the `label` is converted to a torch tensor, why does the image not get converted? How can I fix this issue?
Thanks,
Gunjan
EDIT:
I just checked the shapes, and the types, `batch[image]` is a actually a list of list of tensors. Shape is (1,28,2,28), where `batch_size` is 2. I don't understand why this is happening. Ideally it should be a tensor of shape (2,1,28,28).
EDIT 2:
Inside `prepare_train_features`, the shape of `images[0]` is `torch.Size([1,28,28])`, the conversion is working. However, the output of the `map` is a list of list of list of list. | 299 | Setting to torch format not working with torchvision and MNIST
Hi
I am trying to use `torchvision.transforms` to handle the transformation of the image data in the `mnist` dataset. Assume I have a `transform` variable which contains the `torchvision.transforms` object.
A snippet of what I am trying to do:
```python
def prepare_features(examples):
images = []
labels = []
for example_idx, example in enumerate(examples["image"]):
if transform is not None:
images.append(transform(
np.array(examples["image"][example_idx], dtype=np.uint8)
))
else:
images.append(torch.tensor(np.array(examples["image"][example_idx], dtype=np.uint8)))
labels.append(torch.tensor(examples["label"][example_idx]))
output = {"label":labels, "image":images}
return output
raw_dataset = load_dataset('mnist')
train_dataset = raw_dataset.map(prepare_features, batched=True, batch_size=10000)
train_dataset.set_format("torch",columns=["image","label"])
```
After this, I check the type of the following:
```python
print(type(train_dataset["train"]["label"]))
print(type(train_dataset["train"]["image"][0]))
```
This leads to the following output:
```python
<class 'torch.Tensor'>
<class 'list'>
```
I use `torch.utils.DataLoader` for batches, the type of `batch["train"]["image"]` is also `<class 'list'>`.
I don't understand why only the `label` is converted to a torch tensor, why does the image not get converted? How can I fix this issue?
Thanks,
Gunjan
EDIT:
I just checked the shapes, and the types, `batch[image]` is a actually a list of list of tensors. Shape is (1,28,2,28), where `batch_size` is 2. I don't understand why this is happening. Ideally it should be a tensor of shape (2,1,28,28).
EDIT 2:
Inside `prepare_train_features`, the shape of `images[0]` is `torch.Size([1,28,28])`, the conversion is working. However, the output of the `map` is a list of list of list of list.
# Convert raw tensors to torch format
Strangely, converting to torch tensors works perfectly on `raw_dataset`:
```python
raw_dataset.set_format('torch',columns=['image','label'])
```
Types:
```
Image:
<class 'torch.Tensor'> 60000
<class 'torch.Tensor'> 28
<class 'torch.Tensor'> 28
<class 'torch.Tensor'>
Label:
<class 'torch.Tensor'> 60000
<class 'torch.Tensor'>
```
Using this for transforms:
```python
def prepare_features(examples):
images = []
labels = []
for example_idx, example in enumerate(examples["image"]):
if transform is not None:
images.append(transform(
examples["image"][example_idx].numpy()
))
else:
images.append(examples["image"][example_idx].numpy())
labels.append(examples["label"][example_idx])
output = {"label":labels, "image":images}
return output
```
Inside `prepare_train_features`:
```
Image:
<class 'list'> 10000
<class 'torch.Tensor'> 1
<class 'torch.Tensor'> 28
<class 'torch.Tensor'> 28
<class 'torch.Tensor'>
Label:
<class 'list'> 10000
<class 'torch.Tensor'>
```
After `map`:
```
Image:
<class 'list'> 60000
<class 'list'> 1
<class 'list'> 28
<class 'torch.Tensor'> 28
<class 'torch.Tensor'>
Label:
<class 'torch.Tensor'> 60000
<class 'torch.Tensor'>
```
DataLoader batch:
```
Image:
<class 'list'> 1
<class 'list'> 28
<class 'torch.Tensor'> 2
<class 'torch.Tensor'> 28
<class 'torch.Tensor'>
Label:
<class 'torch.Tensor'> 2
<class 'torch.Tensor'>
```
---
## Using `torch` format:
```
Image:
<class 'list'> 60000
<class 'list'> 1
<class 'list'> 28
<class 'torch.Tensor'> 28
<class 'torch.Tensor'>
Label:
<class 'torch.Tensor'> 60000
<class 'torch.Tensor'>
```
DataLoader batches:
```
Image:
<class 'list'> 1
<class 'list'> 28
<class 'torch.Tensor'> 2
<class 'torch.Tensor'> 28
<class 'torch.Tensor'>
Label:
<class 'torch.Tensor'> 2
<class 'torch.Tensor'>
```
---
## Using the features - `Array3D`:
```
Image:
<class 'list'> 10000
<class 'torch.Tensor'> 1
<class 'torch.Tensor'> 28
<class 'torch.Tensor'> 28
<class 'torch.Tensor'>
Label:
<class 'list'> 10000
<class 'torch.Tensor'>
```
After `map`:
```
Image:
<class 'torch.Tensor'> 60000
<class 'torch.Tensor'> 1
<class 'torch.Tensor'> 28
<class 'torch.Tensor'> 28
<class 'torch.Tensor'>
Label:
<class 'torch.Tensor'> 60000
<class 'torch.Tensor'>
```
After DataLoader `batch`:
```
Image:
<class 'torch.Tensor'> 2
<class 'torch.Tensor'> 1
<class 'torch.Tensor'> 28
<class 'torch.Tensor'> 28
<class 'torch.Tensor'>
Label:
<class 'torch.Tensor'> 2
<class 'torch.Tensor'>
```
The last one works perfectly.
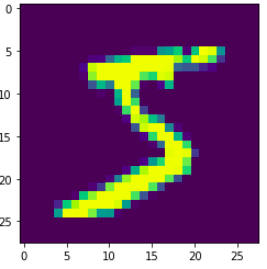
I wonder why this worked, and others didn't.
| [
-0.1273753047,
-0.3511653543,
-0.0179326311,
0.3536090851,
0.4760026336,
0.0887137353,
0.7330292463,
0.3804347813,
0.0655257925,
-0.0380426571,
-0.1111534089,
0.3819510639,
-0.2329690456,
-0.3339343965,
0.0351092033,
-0.56149441,
0.2225798368,
-0.0998275802,
-0.2807980776,
-0.0613473542,
-0.2431338727,
0.0354893208,
-0.2184972465,
-0.1084965691,
-0.5479356647,
0.0440960862,
-0.0195555668,
-0.0765686631,
-0.0622991286,
-0.1391801089,
0.1223466247,
-0.1744965017,
0.4444538355,
0.7012932897,
-0.0001277011,
0.0703017637,
0.3642728031,
-0.1737782061,
0.0499993414,
-0.161013633,
0.0400471762,
-0.2573826909,
0.072776489,
-0.0946833491,
-0.2234944403,
-0.1708453,
0.0959102288,
-0.1790296137,
-0.0281420499,
0.4191690087,
0.0771299899,
0.2790107131,
0.2466612458,
0.1386306435,
0.22781156,
0.5182866454,
-0.273437947,
-0.056117028,
0.2261502147,
0.3754776716,
0.1240048036,
0.5647348166,
-0.2913349569,
-0.0362339392,
0.2669452727,
0.1290050745,
-0.1014554128,
-0.3140053451,
0.0491572432,
0.0894629955,
0.4219443202,
-0.1494392157,
-0.0807788968,
-0.1436638385,
-0.1439783871,
-0.1273806691,
0.040591076,
0.2803193629,
-0.0718411431,
-0.1872384697,
-0.5392424464,
0.3052917719,
-0.1486236155,
0.2131593376,
-0.2805242538,
-0.0034206749,
-0.1381632388,
0.2928087413,
-0.0471191294,
-0.1127126515,
0.193081066,
-0.1234149858,
0.1300754547,
-0.0098257521,
-0.14363195,
-0.0534906499,
-0.3895993531,
-0.3150125742,
-0.2001478821,
-0.1602652222,
0.1664854437,
0.1392757148,
-0.1163955703,
0.1280710101,
0.182633087,
0.2680341601,
0.105782561,
0.3207030296,
-0.0858925655,
-0.2895815372,
-0.0469193235,
0.0877334625,
-0.2962266505,
-0.4357524216,
0.1545345932,
0.3252967596,
0.245221287,
0.0687427744,
0.1298556328,
-0.3239411414,
-0.1089226305,
-0.009586527,
0.0752253011,
0.2850383818,
-0.118679136,
0.2439045608,
0.4804832935,
0.397203058,
-0.1538182348,
0.0695628896,
0.0250724126,
0.0315210149,
-0.4404955208,
-0.2856985331,
0.1534095705,
-0.1177731901,
0.1000702605,
0.0374316536,
0.0464156754,
0.1006964073,
0.073496595,
-0.1858698726,
0.6246293187,
0.1476473957,
-0.1944741011,
0.2767930329,
0.2709067166,
0.5297800303,
-0.3042984605,
0.2565315962,
-0.5095698833,
0.065252535,
-0.0230915733,
-0.0145439208,
0.218655169,
-0.0207218453,
-0.21216245,
0.0158290844,
0.5923122764,
-0.0836781189,
0.2734875381,
-0.5791625381,
0.0949641094,
-0.1913349926,
0.0341524445,
0.0636693314,
-0.2047153264,
-0.0523584448,
0.3863435686,
0.091949828,
0.3154586852,
0.1602820307,
0.0510832109,
0.0798702538,
-0.2253529727,
-0.2350506932,
0.3363234401,
-0.3768563569,
0.0920385197,
-0.0176934246,
0.0938790515,
-0.0638039783,
0.1120189652,
0.3361780643,
0.0326006748,
-0.1280014962,
0.0294486172,
0.1963144988,
-0.1661821902,
0.1402870268,
0.0773596242,
0.1913028955,
0.1933744848,
0.0350064598,
-0.0156837292,
0.2076538354,
-0.2216716707,
0.1775766164,
-0.0542802736,
-0.0747132748,
0.0711774975,
0.0403781086,
-0.3698906004,
-0.0121967876,
-0.0474697649,
0.1864667982,
-0.2331001908,
-0.0487212278,
0.023635203,
0.0822170898,
0.0582790896,
0.0479247868,
0.0049779508,
-0.1919566393,
-0.1822059304,
-0.1390273869,
-0.0032421914,
0.0922106206,
-0.2094768882,
-0.261724323,
-0.0523434915,
-0.1232490093,
0.1783040464,
0.0588377379,
-0.3590368927,
0.0510256998,
0.0192736685,
-0.3653306365,
-0.2013597339,
0.1733922958,
0.2676690817,
-0.0996205956,
-0.042234946,
0.1916034222,
0.0632891431,
0.1146329641,
-0.5482743979,
0.0834657177,
0.1778421402,
-0.2469699979,
0.2043909729,
0.21627976,
0.148533985,
-0.2755034566,
-0.1960351765,
0.4387867153,
0.1604105979,
0.3159126043,
-0.3300259113,
0.1161018088,
-0.0726466849,
-0.0394020006,
-0.1906338334,
-0.0408079401,
-0.2772819102,
0.0730236918,
-0.1318594962,
0.0129819224,
-0.456918478,
-0.0449361987,
0.2962616384,
0.0887638927,
0.1758723557,
-0.0012161626,
-0.3998460472,
0.2133122683,
0.1771266311,
-0.6013534069,
0.2978382111,
-0.0043618386,
0.1892073452,
-0.1050186977,
0.095583491,
-0.1625432819,
0.2484446168,
0.1140421703,
0.0907485485,
-0.1994648427,
0.1418524981,
-0.1079259813,
0.0268464927,
0.0263705309,
-0.1406288743,
-0.2336928695,
-0.3589506149,
0.2713824809,
-0.1661831439,
-0.1345184743,
-0.4018547535,
-0.2621857822,
0.0360562503,
-0.0740049183,
-0.082333751,
0.1507584006,
0.1855680048,
0.1904348731,
0.2634178698,
-0.1818944663,
0.3743163645,
-0.4130294323,
-0.035354875,
-0.0750098228,
-0.286796093,
-0.0991648808,
0.0896559656,
-0.5234106183,
0.0914844051,
-0.2289900333,
-0.0931909382,
-0.2501759827,
0.0135479644,
0.1418222338,
-0.2678501606,
-0.2850028276,
0.3085912764,
0.1939282864,
-0.1424821913,
0.0406621695,
0.2663511932,
0.0103071555,
0.0469012447,
0.1537835598,
-0.0896200985,
0.1165603474,
-0.0632982552,
-0.1767407805,
-0.0966179743,
-0.075061664,
-0.070416607,
-0.0217598137,
0.0633770078,
0.3462583721,
0.372183919,
-0.0898906365,
-0.0520421751,
0.0356090553,
0.0629648119,
-0.3976877034,
0.5225031972,
-0.2261542529,
-0.3608260751,
0.1192018762,
0.0238558389,
0.0651470646,
0.4862686098,
0.060522791,
0.0497318283,
0.0117822066,
-0.0789449736,
0.2525925934,
0.2211973071,
0.3510199785,
-0.0911250561,
0.0191657748,
-0.1647070944,
0.0154164825,
-0.1018714905,
-0.0632553846,
0.6088866591,
0.1850842088,
0.2399428785,
0.0992584452,
0.6996648312,
-0.0611371584,
-0.7380948067,
0.2168068886,
-0.242509678,
0.1921667606,
-0.1181218103,
-0.3539583385,
0.3988562822,
-0.2094198763,
0.0643741786,
-0.0859674439,
-0.024907032,
-0.2391579151,
-0.0902244151,
0.3573616743,
-0.3172068596,
-0.1585739851,
0.4044299424,
-0.1447023898,
0.2763730288,
-0.2313425988,
0.3245900273,
-0.2609262168,
-0.2137925476,
0.0600569248,
0.4487719536,
0.1385134459,
0.0417248979,
-0.3342843056,
-0.2166966051,
-0.1432270408,
0.3568275571,
0.1195773706,
0.5672698021,
-0.0980362967,
-0.0744651258,
0.1015565023,
0.2413697243,
0.8307590485,
0.0455573462,
-0.0904591605,
0.1514422297,
-0.3478347957,
-0.4549783468,
-0.1022293717,
-0.0514177196,
0.0906814784,
0.171690315,
0.1203863472,
-0.0910536647,
-0.1833340377,
-0.0058328966,
0.1955551356,
-0.0551247038,
0.0347188041,
-0.2269114703,
0.058757659,
-0.1296496391,
-0.1552832723,
0.2211825401,
0.0296756551,
0.0051617255,
-0.3081283569,
-0.3262304068,
-0.1266996413,
0.1824819148,
-0.1330122203,
0.4110674262,
0.0992513523,
-0.1780855954,
0.076906763,
0.5561057329,
0.4184041321,
-0.0389392413,
-0.1187734976,
0.0806434527,
0.2931110561,
0.1064323112,
0.3002042174,
0.320822835,
-0.0618730187,
-0.4396643341,
-0.2268486619,
-0.1938148439,
-0.3305326402,
0.3972931504,
0.4080632627,
0.0989354551,
-0.4953554273,
-0.4921705425,
0.3861900568,
0.2937192619,
0.1958300769,
0.2045917213,
-0.5417593122,
-0.4009674489,
0.0781785697,
0.4662418664,
0.8556217551,
-0.037078727,
0.3702827692,
0.0315655693,
0.0108071193,
0.1721023768,
-0.0018886278,
0.4117948115,
-0.3542813957,
0.1392895281,
-0.1648537517,
-0.3125495315,
0.2525228262,
-0.060203135,
-0.2439696491,
0.0425363369,
-0.3494715691,
0.1936195642,
-0.1256936193,
-0.0828530714,
-0.0498264618,
-0.4714174271,
0.1878736466,
0.0154520553,
-0.0825134888,
0.2617389858,
0.1111611798,
-0.1636207998,
-0.3798605204,
0.0616962239,
-0.0762221143,
-0.0150117185,
-0.2459690571,
-0.1632432491,
-0.0243102051,
-0.7298656702,
0.0586798526,
0.4961580336,
0.3345546722,
-0.160566479,
-0.0478368364,
0.1396413594,
0.4438471496,
0.1629004925,
-0.0990172699,
-0.3401843011,
0.2853403986,
0.0973753631,
0.1843320727,
0.1086379662,
0.0681485757,
0.2105205804,
-0.577789247,
0.0372510366,
0.3060290217,
-0.3449506164,
-0.3229845464,
-0.1984502971,
0.0491850227,
0.0381633341,
0.0286184791,
0.124488771,
-0.0389032736,
0.2072432339,
-0.0415120944,
-0.2264618576,
0.1328899711,
0.4523314238,
0.112983726,
-0.3500464261,
0.3907569349,
0.3549591601,
-0.0447779372,
-0.0437441096,
0.4166942239,
0.6353191733,
-0.3456966281,
0.0994976684,
-0.1027166471,
-0.1569870114,
-0.0110735875,
0.5430940986,
-0.2343174964,
-0.1309778541,
-0.2389573902,
-0.0408173315,
-0.4308937788,
0.0108135957,
-0.3162982762,
0.456630528,
-0.0432226621,
0.3627102971,
0.210505724,
-0.1873493046,
-0.1441360116,
-0.2586487532,
0.1723508388,
0.3679335117,
-0.2471824437,
0.2187612951,
-0.1269584745,
-0.1220230311,
-0.0362523533,
0.0260314047,
-0.2497397214,
-0.0333907679,
-0.0576779619,
0.1773060858,
0.0666143522,
-0.1135402918,
-0.0735467896,
-0.1736695915,
-0.1385810077,
-0.2681719065,
-0.037718121,
0.1109212413,
-0.11599949,
0.3531612456,
-0.178366214,
0.2312256396,
0.3186765909,
-0.2700001597,
-0.1026224792,
0.0358713232,
0.2229627371,
0.0600504763,
0.0045319153,
-0.0615568496,
-0.2772366107,
0.1125916764,
0.1652028263,
0.2549746037,
0.2046174854,
-0.0999964103,
0.1091783047,
-0.0438066572,
0.2041842341,
0.1012554616,
-0.1718705595,
-0.050828699,
0.0603729896,
-0.0076234438,
0.1006219834,
0.1449991316,
0.0388691351,
0.1252815872,
-0.0834641606,
0.275578022,
0.0665585697,
0.3113947809,
-0.3705078661,
0.0451425537,
0.4178565443,
-0.2410733104,
0.2350862026,
0.4640147686,
0.0147997756,
0.1930496842,
0.290704608,
0.1093435511,
-0.1187017635,
0.3226963878,
0.3945167661,
0.820430994,
0.5895959139,
0.0837696865,
0.2746027708,
0.0992569551,
-0.1536075622,
-0.2072515339,
0.2358236462,
0.3919682801,
0.0188666489,
0.1856931895,
-0.2407990843,
-0.4871240854,
0.225882113,
0.1217747778,
-0.1946178228,
-0.082223542,
-0.6648060083,
0.0118752681,
0.1358262002,
-0.1866595894,
-0.1797155738,
-0.0501377247,
0.2205908448,
0.2597535551,
-0.1032206789,
-0.1609123051,
-0.1724743992,
0.0691012219,
0.037529368,
-0.007329775,
0.1697572321,
-0.1368460655,
0.1617608517,
0.2448244244,
0.1969081759,
0.284953326,
0.4870801568,
-0.1681717485,
-0.1598939151,
-0.0105530387,
-0.1923659146,
0.0899016932,
0.066711925,
0.031533774,
-0.1532076597,
-0.0149563281,
0.0084625762,
0.0386277921,
0.0314605534,
-0.0940808728,
-0.2182596475,
0.1676249504,
0.3055749238,
-0.3637773693,
-0.1490927637,
-0.0998983905,
-0.2466560602,
-0.3483100832,
-0.2655346692,
0.5327388048,
0.0278958473,
0.1280099303,
0.1421680599,
-0.0421595387,
0.0487859175,
0.1574119478,
-0.121556811,
0.0775766447,
-0.1540937126,
0.1359660923,
-0.0858048201,
0.0319104381,
0.1184747368,
-0.3834479749,
-0.0188493319,
-0.0391645581,
0.2425420135,
0.0646747723,
0.2020902038,
-0.0983472839,
0.4987450838,
0.6146567464,
-0.1336154193,
-0.1414809078,
-0.1304193139,
0.3706512451,
0.0733085498,
-0.0774656981,
0.0219215602,
0.1024274155,
0.0065069334,
-0.3420648277,
-0.2554903924,
-0.0199823231,
0.3041450083,
0.3512826264,
0.0932883546,
0.3203133047,
-0.0192206632,
0.0484100245,
-0.2411341518,
0.0504153185,
-0.1276014,
0.0096705668,
-0.0228027813,
0.5810170174,
-0.224402234,
-0.059342429,
-0.4136501551,
0.4290263653,
0.0905311108,
-0.3388552368,
-0.0805192441,
0.3583999276,
-0.3173208535,
-0.2010883093,
0.226195246,
0.410022229,
0.1513598859,
0.1943574995,
-0.5254407525,
-0.4069116116,
0.4472022951,
-0.4417079389,
-0.5069123507,
0.0290744491,
-0.3133937716,
0.0679039732,
0.4964230061,
-0.4710497558,
-0.1491341293,
0.0015052542,
-0.080827713,
-0.0916047096,
-0.0981937125,
0.2610401213,
0.0151107861,
-0.2518463135,
0.1790072173,
0.1764502972,
-0.0228159856,
-0.4204159677,
-0.2753599882
]
|
https://github.com/huggingface/datasets/issues/2005 | Setting to torch format not working with torchvision and MNIST | Concluding, the way it works right now is:
1. Converting raw dataset to `torch` format.
2. Use the transform and apply using `map`, ensure the returned values are tensors.
3. When mapping, use `features` with `image` being `Array3D` type. | Hi
I am trying to use `torchvision.transforms` to handle the transformation of the image data in the `mnist` dataset. Assume I have a `transform` variable which contains the `torchvision.transforms` object.
A snippet of what I am trying to do:
```python
def prepare_features(examples):
images = []
labels = []
for example_idx, example in enumerate(examples["image"]):
if transform is not None:
images.append(transform(
np.array(examples["image"][example_idx], dtype=np.uint8)
))
else:
images.append(torch.tensor(np.array(examples["image"][example_idx], dtype=np.uint8)))
labels.append(torch.tensor(examples["label"][example_idx]))
output = {"label":labels, "image":images}
return output
raw_dataset = load_dataset('mnist')
train_dataset = raw_dataset.map(prepare_features, batched=True, batch_size=10000)
train_dataset.set_format("torch",columns=["image","label"])
```
After this, I check the type of the following:
```python
print(type(train_dataset["train"]["label"]))
print(type(train_dataset["train"]["image"][0]))
```
This leads to the following output:
```python
<class 'torch.Tensor'>
<class 'list'>
```
I use `torch.utils.DataLoader` for batches, the type of `batch["train"]["image"]` is also `<class 'list'>`.
I don't understand why only the `label` is converted to a torch tensor, why does the image not get converted? How can I fix this issue?
Thanks,
Gunjan
EDIT:
I just checked the shapes, and the types, `batch[image]` is a actually a list of list of tensors. Shape is (1,28,2,28), where `batch_size` is 2. I don't understand why this is happening. Ideally it should be a tensor of shape (2,1,28,28).
EDIT 2:
Inside `prepare_train_features`, the shape of `images[0]` is `torch.Size([1,28,28])`, the conversion is working. However, the output of the `map` is a list of list of list of list. | 39 | Setting to torch format not working with torchvision and MNIST
Hi
I am trying to use `torchvision.transforms` to handle the transformation of the image data in the `mnist` dataset. Assume I have a `transform` variable which contains the `torchvision.transforms` object.
A snippet of what I am trying to do:
```python
def prepare_features(examples):
images = []
labels = []
for example_idx, example in enumerate(examples["image"]):
if transform is not None:
images.append(transform(
np.array(examples["image"][example_idx], dtype=np.uint8)
))
else:
images.append(torch.tensor(np.array(examples["image"][example_idx], dtype=np.uint8)))
labels.append(torch.tensor(examples["label"][example_idx]))
output = {"label":labels, "image":images}
return output
raw_dataset = load_dataset('mnist')
train_dataset = raw_dataset.map(prepare_features, batched=True, batch_size=10000)
train_dataset.set_format("torch",columns=["image","label"])
```
After this, I check the type of the following:
```python
print(type(train_dataset["train"]["label"]))
print(type(train_dataset["train"]["image"][0]))
```
This leads to the following output:
```python
<class 'torch.Tensor'>
<class 'list'>
```
I use `torch.utils.DataLoader` for batches, the type of `batch["train"]["image"]` is also `<class 'list'>`.
I don't understand why only the `label` is converted to a torch tensor, why does the image not get converted? How can I fix this issue?
Thanks,
Gunjan
EDIT:
I just checked the shapes, and the types, `batch[image]` is a actually a list of list of tensors. Shape is (1,28,2,28), where `batch_size` is 2. I don't understand why this is happening. Ideally it should be a tensor of shape (2,1,28,28).
EDIT 2:
Inside `prepare_train_features`, the shape of `images[0]` is `torch.Size([1,28,28])`, the conversion is working. However, the output of the `map` is a list of list of list of list.
Concluding, the way it works right now is:
1. Converting raw dataset to `torch` format.
2. Use the transform and apply using `map`, ensure the returned values are tensors.
3. When mapping, use `features` with `image` being `Array3D` type. | [
-0.1273753047,
-0.3511653543,
-0.0179326311,
0.3536090851,
0.4760026336,
0.0887137353,
0.7330292463,
0.3804347813,
0.0655257925,
-0.0380426571,
-0.1111534089,
0.3819510639,
-0.2329690456,
-0.3339343965,
0.0351092033,
-0.56149441,
0.2225798368,
-0.0998275802,
-0.2807980776,
-0.0613473542,
-0.2431338727,
0.0354893208,
-0.2184972465,
-0.1084965691,
-0.5479356647,
0.0440960862,
-0.0195555668,
-0.0765686631,
-0.0622991286,
-0.1391801089,
0.1223466247,
-0.1744965017,
0.4444538355,
0.7012932897,
-0.0001277011,
0.0703017637,
0.3642728031,
-0.1737782061,
0.0499993414,
-0.161013633,
0.0400471762,
-0.2573826909,
0.072776489,
-0.0946833491,
-0.2234944403,
-0.1708453,
0.0959102288,
-0.1790296137,
-0.0281420499,
0.4191690087,
0.0771299899,
0.2790107131,
0.2466612458,
0.1386306435,
0.22781156,
0.5182866454,
-0.273437947,
-0.056117028,
0.2261502147,
0.3754776716,
0.1240048036,
0.5647348166,
-0.2913349569,
-0.0362339392,
0.2669452727,
0.1290050745,
-0.1014554128,
-0.3140053451,
0.0491572432,
0.0894629955,
0.4219443202,
-0.1494392157,
-0.0807788968,
-0.1436638385,
-0.1439783871,
-0.1273806691,
0.040591076,
0.2803193629,
-0.0718411431,
-0.1872384697,
-0.5392424464,
0.3052917719,
-0.1486236155,
0.2131593376,
-0.2805242538,
-0.0034206749,
-0.1381632388,
0.2928087413,
-0.0471191294,
-0.1127126515,
0.193081066,
-0.1234149858,
0.1300754547,
-0.0098257521,
-0.14363195,
-0.0534906499,
-0.3895993531,
-0.3150125742,
-0.2001478821,
-0.1602652222,
0.1664854437,
0.1392757148,
-0.1163955703,
0.1280710101,
0.182633087,
0.2680341601,
0.105782561,
0.3207030296,
-0.0858925655,
-0.2895815372,
-0.0469193235,
0.0877334625,
-0.2962266505,
-0.4357524216,
0.1545345932,
0.3252967596,
0.245221287,
0.0687427744,
0.1298556328,
-0.3239411414,
-0.1089226305,
-0.009586527,
0.0752253011,
0.2850383818,
-0.118679136,
0.2439045608,
0.4804832935,
0.397203058,
-0.1538182348,
0.0695628896,
0.0250724126,
0.0315210149,
-0.4404955208,
-0.2856985331,
0.1534095705,
-0.1177731901,
0.1000702605,
0.0374316536,
0.0464156754,
0.1006964073,
0.073496595,
-0.1858698726,
0.6246293187,
0.1476473957,
-0.1944741011,
0.2767930329,
0.2709067166,
0.5297800303,
-0.3042984605,
0.2565315962,
-0.5095698833,
0.065252535,
-0.0230915733,
-0.0145439208,
0.218655169,
-0.0207218453,
-0.21216245,
0.0158290844,
0.5923122764,
-0.0836781189,
0.2734875381,
-0.5791625381,
0.0949641094,
-0.1913349926,
0.0341524445,
0.0636693314,
-0.2047153264,
-0.0523584448,
0.3863435686,
0.091949828,
0.3154586852,
0.1602820307,
0.0510832109,
0.0798702538,
-0.2253529727,
-0.2350506932,
0.3363234401,
-0.3768563569,
0.0920385197,
-0.0176934246,
0.0938790515,
-0.0638039783,
0.1120189652,
0.3361780643,
0.0326006748,
-0.1280014962,
0.0294486172,
0.1963144988,
-0.1661821902,
0.1402870268,
0.0773596242,
0.1913028955,
0.1933744848,
0.0350064598,
-0.0156837292,
0.2076538354,
-0.2216716707,
0.1775766164,
-0.0542802736,
-0.0747132748,
0.0711774975,
0.0403781086,
-0.3698906004,
-0.0121967876,
-0.0474697649,
0.1864667982,
-0.2331001908,
-0.0487212278,
0.023635203,
0.0822170898,
0.0582790896,
0.0479247868,
0.0049779508,
-0.1919566393,
-0.1822059304,
-0.1390273869,
-0.0032421914,
0.0922106206,
-0.2094768882,
-0.261724323,
-0.0523434915,
-0.1232490093,
0.1783040464,
0.0588377379,
-0.3590368927,
0.0510256998,
0.0192736685,
-0.3653306365,
-0.2013597339,
0.1733922958,
0.2676690817,
-0.0996205956,
-0.042234946,
0.1916034222,
0.0632891431,
0.1146329641,
-0.5482743979,
0.0834657177,
0.1778421402,
-0.2469699979,
0.2043909729,
0.21627976,
0.148533985,
-0.2755034566,
-0.1960351765,
0.4387867153,
0.1604105979,
0.3159126043,
-0.3300259113,
0.1161018088,
-0.0726466849,
-0.0394020006,
-0.1906338334,
-0.0408079401,
-0.2772819102,
0.0730236918,
-0.1318594962,
0.0129819224,
-0.456918478,
-0.0449361987,
0.2962616384,
0.0887638927,
0.1758723557,
-0.0012161626,
-0.3998460472,
0.2133122683,
0.1771266311,
-0.6013534069,
0.2978382111,
-0.0043618386,
0.1892073452,
-0.1050186977,
0.095583491,
-0.1625432819,
0.2484446168,
0.1140421703,
0.0907485485,
-0.1994648427,
0.1418524981,
-0.1079259813,
0.0268464927,
0.0263705309,
-0.1406288743,
-0.2336928695,
-0.3589506149,
0.2713824809,
-0.1661831439,
-0.1345184743,
-0.4018547535,
-0.2621857822,
0.0360562503,
-0.0740049183,
-0.082333751,
0.1507584006,
0.1855680048,
0.1904348731,
0.2634178698,
-0.1818944663,
0.3743163645,
-0.4130294323,
-0.035354875,
-0.0750098228,
-0.286796093,
-0.0991648808,
0.0896559656,
-0.5234106183,
0.0914844051,
-0.2289900333,
-0.0931909382,
-0.2501759827,
0.0135479644,
0.1418222338,
-0.2678501606,
-0.2850028276,
0.3085912764,
0.1939282864,
-0.1424821913,
0.0406621695,
0.2663511932,
0.0103071555,
0.0469012447,
0.1537835598,
-0.0896200985,
0.1165603474,
-0.0632982552,
-0.1767407805,
-0.0966179743,
-0.075061664,
-0.070416607,
-0.0217598137,
0.0633770078,
0.3462583721,
0.372183919,
-0.0898906365,
-0.0520421751,
0.0356090553,
0.0629648119,
-0.3976877034,
0.5225031972,
-0.2261542529,
-0.3608260751,
0.1192018762,
0.0238558389,
0.0651470646,
0.4862686098,
0.060522791,
0.0497318283,
0.0117822066,
-0.0789449736,
0.2525925934,
0.2211973071,
0.3510199785,
-0.0911250561,
0.0191657748,
-0.1647070944,
0.0154164825,
-0.1018714905,
-0.0632553846,
0.6088866591,
0.1850842088,
0.2399428785,
0.0992584452,
0.6996648312,
-0.0611371584,
-0.7380948067,
0.2168068886,
-0.242509678,
0.1921667606,
-0.1181218103,
-0.3539583385,
0.3988562822,
-0.2094198763,
0.0643741786,
-0.0859674439,
-0.024907032,
-0.2391579151,
-0.0902244151,
0.3573616743,
-0.3172068596,
-0.1585739851,
0.4044299424,
-0.1447023898,
0.2763730288,
-0.2313425988,
0.3245900273,
-0.2609262168,
-0.2137925476,
0.0600569248,
0.4487719536,
0.1385134459,
0.0417248979,
-0.3342843056,
-0.2166966051,
-0.1432270408,
0.3568275571,
0.1195773706,
0.5672698021,
-0.0980362967,
-0.0744651258,
0.1015565023,
0.2413697243,
0.8307590485,
0.0455573462,
-0.0904591605,
0.1514422297,
-0.3478347957,
-0.4549783468,
-0.1022293717,
-0.0514177196,
0.0906814784,
0.171690315,
0.1203863472,
-0.0910536647,
-0.1833340377,
-0.0058328966,
0.1955551356,
-0.0551247038,
0.0347188041,
-0.2269114703,
0.058757659,
-0.1296496391,
-0.1552832723,
0.2211825401,
0.0296756551,
0.0051617255,
-0.3081283569,
-0.3262304068,
-0.1266996413,
0.1824819148,
-0.1330122203,
0.4110674262,
0.0992513523,
-0.1780855954,
0.076906763,
0.5561057329,
0.4184041321,
-0.0389392413,
-0.1187734976,
0.0806434527,
0.2931110561,
0.1064323112,
0.3002042174,
0.320822835,
-0.0618730187,
-0.4396643341,
-0.2268486619,
-0.1938148439,
-0.3305326402,
0.3972931504,
0.4080632627,
0.0989354551,
-0.4953554273,
-0.4921705425,
0.3861900568,
0.2937192619,
0.1958300769,
0.2045917213,
-0.5417593122,
-0.4009674489,
0.0781785697,
0.4662418664,
0.8556217551,
-0.037078727,
0.3702827692,
0.0315655693,
0.0108071193,
0.1721023768,
-0.0018886278,
0.4117948115,
-0.3542813957,
0.1392895281,
-0.1648537517,
-0.3125495315,
0.2525228262,
-0.060203135,
-0.2439696491,
0.0425363369,
-0.3494715691,
0.1936195642,
-0.1256936193,
-0.0828530714,
-0.0498264618,
-0.4714174271,
0.1878736466,
0.0154520553,
-0.0825134888,
0.2617389858,
0.1111611798,
-0.1636207998,
-0.3798605204,
0.0616962239,
-0.0762221143,
-0.0150117185,
-0.2459690571,
-0.1632432491,
-0.0243102051,
-0.7298656702,
0.0586798526,
0.4961580336,
0.3345546722,
-0.160566479,
-0.0478368364,
0.1396413594,
0.4438471496,
0.1629004925,
-0.0990172699,
-0.3401843011,
0.2853403986,
0.0973753631,
0.1843320727,
0.1086379662,
0.0681485757,
0.2105205804,
-0.577789247,
0.0372510366,
0.3060290217,
-0.3449506164,
-0.3229845464,
-0.1984502971,
0.0491850227,
0.0381633341,
0.0286184791,
0.124488771,
-0.0389032736,
0.2072432339,
-0.0415120944,
-0.2264618576,
0.1328899711,
0.4523314238,
0.112983726,
-0.3500464261,
0.3907569349,
0.3549591601,
-0.0447779372,
-0.0437441096,
0.4166942239,
0.6353191733,
-0.3456966281,
0.0994976684,
-0.1027166471,
-0.1569870114,
-0.0110735875,
0.5430940986,
-0.2343174964,
-0.1309778541,
-0.2389573902,
-0.0408173315,
-0.4308937788,
0.0108135957,
-0.3162982762,
0.456630528,
-0.0432226621,
0.3627102971,
0.210505724,
-0.1873493046,
-0.1441360116,
-0.2586487532,
0.1723508388,
0.3679335117,
-0.2471824437,
0.2187612951,
-0.1269584745,
-0.1220230311,
-0.0362523533,
0.0260314047,
-0.2497397214,
-0.0333907679,
-0.0576779619,
0.1773060858,
0.0666143522,
-0.1135402918,
-0.0735467896,
-0.1736695915,
-0.1385810077,
-0.2681719065,
-0.037718121,
0.1109212413,
-0.11599949,
0.3531612456,
-0.178366214,
0.2312256396,
0.3186765909,
-0.2700001597,
-0.1026224792,
0.0358713232,
0.2229627371,
0.0600504763,
0.0045319153,
-0.0615568496,
-0.2772366107,
0.1125916764,
0.1652028263,
0.2549746037,
0.2046174854,
-0.0999964103,
0.1091783047,
-0.0438066572,
0.2041842341,
0.1012554616,
-0.1718705595,
-0.050828699,
0.0603729896,
-0.0076234438,
0.1006219834,
0.1449991316,
0.0388691351,
0.1252815872,
-0.0834641606,
0.275578022,
0.0665585697,
0.3113947809,
-0.3705078661,
0.0451425537,
0.4178565443,
-0.2410733104,
0.2350862026,
0.4640147686,
0.0147997756,
0.1930496842,
0.290704608,
0.1093435511,
-0.1187017635,
0.3226963878,
0.3945167661,
0.820430994,
0.5895959139,
0.0837696865,
0.2746027708,
0.0992569551,
-0.1536075622,
-0.2072515339,
0.2358236462,
0.3919682801,
0.0188666489,
0.1856931895,
-0.2407990843,
-0.4871240854,
0.225882113,
0.1217747778,
-0.1946178228,
-0.082223542,
-0.6648060083,
0.0118752681,
0.1358262002,
-0.1866595894,
-0.1797155738,
-0.0501377247,
0.2205908448,
0.2597535551,
-0.1032206789,
-0.1609123051,
-0.1724743992,
0.0691012219,
0.037529368,
-0.007329775,
0.1697572321,
-0.1368460655,
0.1617608517,
0.2448244244,
0.1969081759,
0.284953326,
0.4870801568,
-0.1681717485,
-0.1598939151,
-0.0105530387,
-0.1923659146,
0.0899016932,
0.066711925,
0.031533774,
-0.1532076597,
-0.0149563281,
0.0084625762,
0.0386277921,
0.0314605534,
-0.0940808728,
-0.2182596475,
0.1676249504,
0.3055749238,
-0.3637773693,
-0.1490927637,
-0.0998983905,
-0.2466560602,
-0.3483100832,
-0.2655346692,
0.5327388048,
0.0278958473,
0.1280099303,
0.1421680599,
-0.0421595387,
0.0487859175,
0.1574119478,
-0.121556811,
0.0775766447,
-0.1540937126,
0.1359660923,
-0.0858048201,
0.0319104381,
0.1184747368,
-0.3834479749,
-0.0188493319,
-0.0391645581,
0.2425420135,
0.0646747723,
0.2020902038,
-0.0983472839,
0.4987450838,
0.6146567464,
-0.1336154193,
-0.1414809078,
-0.1304193139,
0.3706512451,
0.0733085498,
-0.0774656981,
0.0219215602,
0.1024274155,
0.0065069334,
-0.3420648277,
-0.2554903924,
-0.0199823231,
0.3041450083,
0.3512826264,
0.0932883546,
0.3203133047,
-0.0192206632,
0.0484100245,
-0.2411341518,
0.0504153185,
-0.1276014,
0.0096705668,
-0.0228027813,
0.5810170174,
-0.224402234,
-0.059342429,
-0.4136501551,
0.4290263653,
0.0905311108,
-0.3388552368,
-0.0805192441,
0.3583999276,
-0.3173208535,
-0.2010883093,
0.226195246,
0.410022229,
0.1513598859,
0.1943574995,
-0.5254407525,
-0.4069116116,
0.4472022951,
-0.4417079389,
-0.5069123507,
0.0290744491,
-0.3133937716,
0.0679039732,
0.4964230061,
-0.4710497558,
-0.1491341293,
0.0015052542,
-0.080827713,
-0.0916047096,
-0.0981937125,
0.2610401213,
0.0151107861,
-0.2518463135,
0.1790072173,
0.1764502972,
-0.0228159856,
-0.4204159677,
-0.2753599882
]
|
https://github.com/huggingface/datasets/issues/2005 | Setting to torch format not working with torchvision and MNIST | What the dataset returns depends on the feature type.
For a feature type that is Sequence(Sequence(Sequence(Value("uint8")))), a dataset formatted as "torch" return lists of lists of tensors. This is because the lists lengths may vary.
For a feature type that is Array3D on the other hand it returns one tensor. This is because the size of the tensor is fixed and defined bu the Array3D type. | Hi
I am trying to use `torchvision.transforms` to handle the transformation of the image data in the `mnist` dataset. Assume I have a `transform` variable which contains the `torchvision.transforms` object.
A snippet of what I am trying to do:
```python
def prepare_features(examples):
images = []
labels = []
for example_idx, example in enumerate(examples["image"]):
if transform is not None:
images.append(transform(
np.array(examples["image"][example_idx], dtype=np.uint8)
))
else:
images.append(torch.tensor(np.array(examples["image"][example_idx], dtype=np.uint8)))
labels.append(torch.tensor(examples["label"][example_idx]))
output = {"label":labels, "image":images}
return output
raw_dataset = load_dataset('mnist')
train_dataset = raw_dataset.map(prepare_features, batched=True, batch_size=10000)
train_dataset.set_format("torch",columns=["image","label"])
```
After this, I check the type of the following:
```python
print(type(train_dataset["train"]["label"]))
print(type(train_dataset["train"]["image"][0]))
```
This leads to the following output:
```python
<class 'torch.Tensor'>
<class 'list'>
```
I use `torch.utils.DataLoader` for batches, the type of `batch["train"]["image"]` is also `<class 'list'>`.
I don't understand why only the `label` is converted to a torch tensor, why does the image not get converted? How can I fix this issue?
Thanks,
Gunjan
EDIT:
I just checked the shapes, and the types, `batch[image]` is a actually a list of list of tensors. Shape is (1,28,2,28), where `batch_size` is 2. I don't understand why this is happening. Ideally it should be a tensor of shape (2,1,28,28).
EDIT 2:
Inside `prepare_train_features`, the shape of `images[0]` is `torch.Size([1,28,28])`, the conversion is working. However, the output of the `map` is a list of list of list of list. | 66 | Setting to torch format not working with torchvision and MNIST
Hi
I am trying to use `torchvision.transforms` to handle the transformation of the image data in the `mnist` dataset. Assume I have a `transform` variable which contains the `torchvision.transforms` object.
A snippet of what I am trying to do:
```python
def prepare_features(examples):
images = []
labels = []
for example_idx, example in enumerate(examples["image"]):
if transform is not None:
images.append(transform(
np.array(examples["image"][example_idx], dtype=np.uint8)
))
else:
images.append(torch.tensor(np.array(examples["image"][example_idx], dtype=np.uint8)))
labels.append(torch.tensor(examples["label"][example_idx]))
output = {"label":labels, "image":images}
return output
raw_dataset = load_dataset('mnist')
train_dataset = raw_dataset.map(prepare_features, batched=True, batch_size=10000)
train_dataset.set_format("torch",columns=["image","label"])
```
After this, I check the type of the following:
```python
print(type(train_dataset["train"]["label"]))
print(type(train_dataset["train"]["image"][0]))
```
This leads to the following output:
```python
<class 'torch.Tensor'>
<class 'list'>
```
I use `torch.utils.DataLoader` for batches, the type of `batch["train"]["image"]` is also `<class 'list'>`.
I don't understand why only the `label` is converted to a torch tensor, why does the image not get converted? How can I fix this issue?
Thanks,
Gunjan
EDIT:
I just checked the shapes, and the types, `batch[image]` is a actually a list of list of tensors. Shape is (1,28,2,28), where `batch_size` is 2. I don't understand why this is happening. Ideally it should be a tensor of shape (2,1,28,28).
EDIT 2:
Inside `prepare_train_features`, the shape of `images[0]` is `torch.Size([1,28,28])`, the conversion is working. However, the output of the `map` is a list of list of list of list.
What the dataset returns depends on the feature type.
For a feature type that is Sequence(Sequence(Sequence(Value("uint8")))), a dataset formatted as "torch" return lists of lists of tensors. This is because the lists lengths may vary.
For a feature type that is Array3D on the other hand it returns one tensor. This is because the size of the tensor is fixed and defined bu the Array3D type. | [
-0.1273753047,
-0.3511653543,
-0.0179326311,
0.3536090851,
0.4760026336,
0.0887137353,
0.7330292463,
0.3804347813,
0.0655257925,
-0.0380426571,
-0.1111534089,
0.3819510639,
-0.2329690456,
-0.3339343965,
0.0351092033,
-0.56149441,
0.2225798368,
-0.0998275802,
-0.2807980776,
-0.0613473542,
-0.2431338727,
0.0354893208,
-0.2184972465,
-0.1084965691,
-0.5479356647,
0.0440960862,
-0.0195555668,
-0.0765686631,
-0.0622991286,
-0.1391801089,
0.1223466247,
-0.1744965017,
0.4444538355,
0.7012932897,
-0.0001277011,
0.0703017637,
0.3642728031,
-0.1737782061,
0.0499993414,
-0.161013633,
0.0400471762,
-0.2573826909,
0.072776489,
-0.0946833491,
-0.2234944403,
-0.1708453,
0.0959102288,
-0.1790296137,
-0.0281420499,
0.4191690087,
0.0771299899,
0.2790107131,
0.2466612458,
0.1386306435,
0.22781156,
0.5182866454,
-0.273437947,
-0.056117028,
0.2261502147,
0.3754776716,
0.1240048036,
0.5647348166,
-0.2913349569,
-0.0362339392,
0.2669452727,
0.1290050745,
-0.1014554128,
-0.3140053451,
0.0491572432,
0.0894629955,
0.4219443202,
-0.1494392157,
-0.0807788968,
-0.1436638385,
-0.1439783871,
-0.1273806691,
0.040591076,
0.2803193629,
-0.0718411431,
-0.1872384697,
-0.5392424464,
0.3052917719,
-0.1486236155,
0.2131593376,
-0.2805242538,
-0.0034206749,
-0.1381632388,
0.2928087413,
-0.0471191294,
-0.1127126515,
0.193081066,
-0.1234149858,
0.1300754547,
-0.0098257521,
-0.14363195,
-0.0534906499,
-0.3895993531,
-0.3150125742,
-0.2001478821,
-0.1602652222,
0.1664854437,
0.1392757148,
-0.1163955703,
0.1280710101,
0.182633087,
0.2680341601,
0.105782561,
0.3207030296,
-0.0858925655,
-0.2895815372,
-0.0469193235,
0.0877334625,
-0.2962266505,
-0.4357524216,
0.1545345932,
0.3252967596,
0.245221287,
0.0687427744,
0.1298556328,
-0.3239411414,
-0.1089226305,
-0.009586527,
0.0752253011,
0.2850383818,
-0.118679136,
0.2439045608,
0.4804832935,
0.397203058,
-0.1538182348,
0.0695628896,
0.0250724126,
0.0315210149,
-0.4404955208,
-0.2856985331,
0.1534095705,
-0.1177731901,
0.1000702605,
0.0374316536,
0.0464156754,
0.1006964073,
0.073496595,
-0.1858698726,
0.6246293187,
0.1476473957,
-0.1944741011,
0.2767930329,
0.2709067166,
0.5297800303,
-0.3042984605,
0.2565315962,
-0.5095698833,
0.065252535,
-0.0230915733,
-0.0145439208,
0.218655169,
-0.0207218453,
-0.21216245,
0.0158290844,
0.5923122764,
-0.0836781189,
0.2734875381,
-0.5791625381,
0.0949641094,
-0.1913349926,
0.0341524445,
0.0636693314,
-0.2047153264,
-0.0523584448,
0.3863435686,
0.091949828,
0.3154586852,
0.1602820307,
0.0510832109,
0.0798702538,
-0.2253529727,
-0.2350506932,
0.3363234401,
-0.3768563569,
0.0920385197,
-0.0176934246,
0.0938790515,
-0.0638039783,
0.1120189652,
0.3361780643,
0.0326006748,
-0.1280014962,
0.0294486172,
0.1963144988,
-0.1661821902,
0.1402870268,
0.0773596242,
0.1913028955,
0.1933744848,
0.0350064598,
-0.0156837292,
0.2076538354,
-0.2216716707,
0.1775766164,
-0.0542802736,
-0.0747132748,
0.0711774975,
0.0403781086,
-0.3698906004,
-0.0121967876,
-0.0474697649,
0.1864667982,
-0.2331001908,
-0.0487212278,
0.023635203,
0.0822170898,
0.0582790896,
0.0479247868,
0.0049779508,
-0.1919566393,
-0.1822059304,
-0.1390273869,
-0.0032421914,
0.0922106206,
-0.2094768882,
-0.261724323,
-0.0523434915,
-0.1232490093,
0.1783040464,
0.0588377379,
-0.3590368927,
0.0510256998,
0.0192736685,
-0.3653306365,
-0.2013597339,
0.1733922958,
0.2676690817,
-0.0996205956,
-0.042234946,
0.1916034222,
0.0632891431,
0.1146329641,
-0.5482743979,
0.0834657177,
0.1778421402,
-0.2469699979,
0.2043909729,
0.21627976,
0.148533985,
-0.2755034566,
-0.1960351765,
0.4387867153,
0.1604105979,
0.3159126043,
-0.3300259113,
0.1161018088,
-0.0726466849,
-0.0394020006,
-0.1906338334,
-0.0408079401,
-0.2772819102,
0.0730236918,
-0.1318594962,
0.0129819224,
-0.456918478,
-0.0449361987,
0.2962616384,
0.0887638927,
0.1758723557,
-0.0012161626,
-0.3998460472,
0.2133122683,
0.1771266311,
-0.6013534069,
0.2978382111,
-0.0043618386,
0.1892073452,
-0.1050186977,
0.095583491,
-0.1625432819,
0.2484446168,
0.1140421703,
0.0907485485,
-0.1994648427,
0.1418524981,
-0.1079259813,
0.0268464927,
0.0263705309,
-0.1406288743,
-0.2336928695,
-0.3589506149,
0.2713824809,
-0.1661831439,
-0.1345184743,
-0.4018547535,
-0.2621857822,
0.0360562503,
-0.0740049183,
-0.082333751,
0.1507584006,
0.1855680048,
0.1904348731,
0.2634178698,
-0.1818944663,
0.3743163645,
-0.4130294323,
-0.035354875,
-0.0750098228,
-0.286796093,
-0.0991648808,
0.0896559656,
-0.5234106183,
0.0914844051,
-0.2289900333,
-0.0931909382,
-0.2501759827,
0.0135479644,
0.1418222338,
-0.2678501606,
-0.2850028276,
0.3085912764,
0.1939282864,
-0.1424821913,
0.0406621695,
0.2663511932,
0.0103071555,
0.0469012447,
0.1537835598,
-0.0896200985,
0.1165603474,
-0.0632982552,
-0.1767407805,
-0.0966179743,
-0.075061664,
-0.070416607,
-0.0217598137,
0.0633770078,
0.3462583721,
0.372183919,
-0.0898906365,
-0.0520421751,
0.0356090553,
0.0629648119,
-0.3976877034,
0.5225031972,
-0.2261542529,
-0.3608260751,
0.1192018762,
0.0238558389,
0.0651470646,
0.4862686098,
0.060522791,
0.0497318283,
0.0117822066,
-0.0789449736,
0.2525925934,
0.2211973071,
0.3510199785,
-0.0911250561,
0.0191657748,
-0.1647070944,
0.0154164825,
-0.1018714905,
-0.0632553846,
0.6088866591,
0.1850842088,
0.2399428785,
0.0992584452,
0.6996648312,
-0.0611371584,
-0.7380948067,
0.2168068886,
-0.242509678,
0.1921667606,
-0.1181218103,
-0.3539583385,
0.3988562822,
-0.2094198763,
0.0643741786,
-0.0859674439,
-0.024907032,
-0.2391579151,
-0.0902244151,
0.3573616743,
-0.3172068596,
-0.1585739851,
0.4044299424,
-0.1447023898,
0.2763730288,
-0.2313425988,
0.3245900273,
-0.2609262168,
-0.2137925476,
0.0600569248,
0.4487719536,
0.1385134459,
0.0417248979,
-0.3342843056,
-0.2166966051,
-0.1432270408,
0.3568275571,
0.1195773706,
0.5672698021,
-0.0980362967,
-0.0744651258,
0.1015565023,
0.2413697243,
0.8307590485,
0.0455573462,
-0.0904591605,
0.1514422297,
-0.3478347957,
-0.4549783468,
-0.1022293717,
-0.0514177196,
0.0906814784,
0.171690315,
0.1203863472,
-0.0910536647,
-0.1833340377,
-0.0058328966,
0.1955551356,
-0.0551247038,
0.0347188041,
-0.2269114703,
0.058757659,
-0.1296496391,
-0.1552832723,
0.2211825401,
0.0296756551,
0.0051617255,
-0.3081283569,
-0.3262304068,
-0.1266996413,
0.1824819148,
-0.1330122203,
0.4110674262,
0.0992513523,
-0.1780855954,
0.076906763,
0.5561057329,
0.4184041321,
-0.0389392413,
-0.1187734976,
0.0806434527,
0.2931110561,
0.1064323112,
0.3002042174,
0.320822835,
-0.0618730187,
-0.4396643341,
-0.2268486619,
-0.1938148439,
-0.3305326402,
0.3972931504,
0.4080632627,
0.0989354551,
-0.4953554273,
-0.4921705425,
0.3861900568,
0.2937192619,
0.1958300769,
0.2045917213,
-0.5417593122,
-0.4009674489,
0.0781785697,
0.4662418664,
0.8556217551,
-0.037078727,
0.3702827692,
0.0315655693,
0.0108071193,
0.1721023768,
-0.0018886278,
0.4117948115,
-0.3542813957,
0.1392895281,
-0.1648537517,
-0.3125495315,
0.2525228262,
-0.060203135,
-0.2439696491,
0.0425363369,
-0.3494715691,
0.1936195642,
-0.1256936193,
-0.0828530714,
-0.0498264618,
-0.4714174271,
0.1878736466,
0.0154520553,
-0.0825134888,
0.2617389858,
0.1111611798,
-0.1636207998,
-0.3798605204,
0.0616962239,
-0.0762221143,
-0.0150117185,
-0.2459690571,
-0.1632432491,
-0.0243102051,
-0.7298656702,
0.0586798526,
0.4961580336,
0.3345546722,
-0.160566479,
-0.0478368364,
0.1396413594,
0.4438471496,
0.1629004925,
-0.0990172699,
-0.3401843011,
0.2853403986,
0.0973753631,
0.1843320727,
0.1086379662,
0.0681485757,
0.2105205804,
-0.577789247,
0.0372510366,
0.3060290217,
-0.3449506164,
-0.3229845464,
-0.1984502971,
0.0491850227,
0.0381633341,
0.0286184791,
0.124488771,
-0.0389032736,
0.2072432339,
-0.0415120944,
-0.2264618576,
0.1328899711,
0.4523314238,
0.112983726,
-0.3500464261,
0.3907569349,
0.3549591601,
-0.0447779372,
-0.0437441096,
0.4166942239,
0.6353191733,
-0.3456966281,
0.0994976684,
-0.1027166471,
-0.1569870114,
-0.0110735875,
0.5430940986,
-0.2343174964,
-0.1309778541,
-0.2389573902,
-0.0408173315,
-0.4308937788,
0.0108135957,
-0.3162982762,
0.456630528,
-0.0432226621,
0.3627102971,
0.210505724,
-0.1873493046,
-0.1441360116,
-0.2586487532,
0.1723508388,
0.3679335117,
-0.2471824437,
0.2187612951,
-0.1269584745,
-0.1220230311,
-0.0362523533,
0.0260314047,
-0.2497397214,
-0.0333907679,
-0.0576779619,
0.1773060858,
0.0666143522,
-0.1135402918,
-0.0735467896,
-0.1736695915,
-0.1385810077,
-0.2681719065,
-0.037718121,
0.1109212413,
-0.11599949,
0.3531612456,
-0.178366214,
0.2312256396,
0.3186765909,
-0.2700001597,
-0.1026224792,
0.0358713232,
0.2229627371,
0.0600504763,
0.0045319153,
-0.0615568496,
-0.2772366107,
0.1125916764,
0.1652028263,
0.2549746037,
0.2046174854,
-0.0999964103,
0.1091783047,
-0.0438066572,
0.2041842341,
0.1012554616,
-0.1718705595,
-0.050828699,
0.0603729896,
-0.0076234438,
0.1006219834,
0.1449991316,
0.0388691351,
0.1252815872,
-0.0834641606,
0.275578022,
0.0665585697,
0.3113947809,
-0.3705078661,
0.0451425537,
0.4178565443,
-0.2410733104,
0.2350862026,
0.4640147686,
0.0147997756,
0.1930496842,
0.290704608,
0.1093435511,
-0.1187017635,
0.3226963878,
0.3945167661,
0.820430994,
0.5895959139,
0.0837696865,
0.2746027708,
0.0992569551,
-0.1536075622,
-0.2072515339,
0.2358236462,
0.3919682801,
0.0188666489,
0.1856931895,
-0.2407990843,
-0.4871240854,
0.225882113,
0.1217747778,
-0.1946178228,
-0.082223542,
-0.6648060083,
0.0118752681,
0.1358262002,
-0.1866595894,
-0.1797155738,
-0.0501377247,
0.2205908448,
0.2597535551,
-0.1032206789,
-0.1609123051,
-0.1724743992,
0.0691012219,
0.037529368,
-0.007329775,
0.1697572321,
-0.1368460655,
0.1617608517,
0.2448244244,
0.1969081759,
0.284953326,
0.4870801568,
-0.1681717485,
-0.1598939151,
-0.0105530387,
-0.1923659146,
0.0899016932,
0.066711925,
0.031533774,
-0.1532076597,
-0.0149563281,
0.0084625762,
0.0386277921,
0.0314605534,
-0.0940808728,
-0.2182596475,
0.1676249504,
0.3055749238,
-0.3637773693,
-0.1490927637,
-0.0998983905,
-0.2466560602,
-0.3483100832,
-0.2655346692,
0.5327388048,
0.0278958473,
0.1280099303,
0.1421680599,
-0.0421595387,
0.0487859175,
0.1574119478,
-0.121556811,
0.0775766447,
-0.1540937126,
0.1359660923,
-0.0858048201,
0.0319104381,
0.1184747368,
-0.3834479749,
-0.0188493319,
-0.0391645581,
0.2425420135,
0.0646747723,
0.2020902038,
-0.0983472839,
0.4987450838,
0.6146567464,
-0.1336154193,
-0.1414809078,
-0.1304193139,
0.3706512451,
0.0733085498,
-0.0774656981,
0.0219215602,
0.1024274155,
0.0065069334,
-0.3420648277,
-0.2554903924,
-0.0199823231,
0.3041450083,
0.3512826264,
0.0932883546,
0.3203133047,
-0.0192206632,
0.0484100245,
-0.2411341518,
0.0504153185,
-0.1276014,
0.0096705668,
-0.0228027813,
0.5810170174,
-0.224402234,
-0.059342429,
-0.4136501551,
0.4290263653,
0.0905311108,
-0.3388552368,
-0.0805192441,
0.3583999276,
-0.3173208535,
-0.2010883093,
0.226195246,
0.410022229,
0.1513598859,
0.1943574995,
-0.5254407525,
-0.4069116116,
0.4472022951,
-0.4417079389,
-0.5069123507,
0.0290744491,
-0.3133937716,
0.0679039732,
0.4964230061,
-0.4710497558,
-0.1491341293,
0.0015052542,
-0.080827713,
-0.0916047096,
-0.0981937125,
0.2610401213,
0.0151107861,
-0.2518463135,
0.1790072173,
0.1764502972,
-0.0228159856,
-0.4204159677,
-0.2753599882
]
|
https://github.com/huggingface/datasets/issues/2005 | Setting to torch format not working with torchvision and MNIST | Okay, that makes sense.
Raw images are list of Array2D, hence we get a single tensor when `set_format` is used. But, why should I need to convert the raw images to `torch` format when `map` does this internally?
Using `Array3D` did not work with `map` when raw images weren't `set_format`ted to torch type. | Hi
I am trying to use `torchvision.transforms` to handle the transformation of the image data in the `mnist` dataset. Assume I have a `transform` variable which contains the `torchvision.transforms` object.
A snippet of what I am trying to do:
```python
def prepare_features(examples):
images = []
labels = []
for example_idx, example in enumerate(examples["image"]):
if transform is not None:
images.append(transform(
np.array(examples["image"][example_idx], dtype=np.uint8)
))
else:
images.append(torch.tensor(np.array(examples["image"][example_idx], dtype=np.uint8)))
labels.append(torch.tensor(examples["label"][example_idx]))
output = {"label":labels, "image":images}
return output
raw_dataset = load_dataset('mnist')
train_dataset = raw_dataset.map(prepare_features, batched=True, batch_size=10000)
train_dataset.set_format("torch",columns=["image","label"])
```
After this, I check the type of the following:
```python
print(type(train_dataset["train"]["label"]))
print(type(train_dataset["train"]["image"][0]))
```
This leads to the following output:
```python
<class 'torch.Tensor'>
<class 'list'>
```
I use `torch.utils.DataLoader` for batches, the type of `batch["train"]["image"]` is also `<class 'list'>`.
I don't understand why only the `label` is converted to a torch tensor, why does the image not get converted? How can I fix this issue?
Thanks,
Gunjan
EDIT:
I just checked the shapes, and the types, `batch[image]` is a actually a list of list of tensors. Shape is (1,28,2,28), where `batch_size` is 2. I don't understand why this is happening. Ideally it should be a tensor of shape (2,1,28,28).
EDIT 2:
Inside `prepare_train_features`, the shape of `images[0]` is `torch.Size([1,28,28])`, the conversion is working. However, the output of the `map` is a list of list of list of list. | 53 | Setting to torch format not working with torchvision and MNIST
Hi
I am trying to use `torchvision.transforms` to handle the transformation of the image data in the `mnist` dataset. Assume I have a `transform` variable which contains the `torchvision.transforms` object.
A snippet of what I am trying to do:
```python
def prepare_features(examples):
images = []
labels = []
for example_idx, example in enumerate(examples["image"]):
if transform is not None:
images.append(transform(
np.array(examples["image"][example_idx], dtype=np.uint8)
))
else:
images.append(torch.tensor(np.array(examples["image"][example_idx], dtype=np.uint8)))
labels.append(torch.tensor(examples["label"][example_idx]))
output = {"label":labels, "image":images}
return output
raw_dataset = load_dataset('mnist')
train_dataset = raw_dataset.map(prepare_features, batched=True, batch_size=10000)
train_dataset.set_format("torch",columns=["image","label"])
```
After this, I check the type of the following:
```python
print(type(train_dataset["train"]["label"]))
print(type(train_dataset["train"]["image"][0]))
```
This leads to the following output:
```python
<class 'torch.Tensor'>
<class 'list'>
```
I use `torch.utils.DataLoader` for batches, the type of `batch["train"]["image"]` is also `<class 'list'>`.
I don't understand why only the `label` is converted to a torch tensor, why does the image not get converted? How can I fix this issue?
Thanks,
Gunjan
EDIT:
I just checked the shapes, and the types, `batch[image]` is a actually a list of list of tensors. Shape is (1,28,2,28), where `batch_size` is 2. I don't understand why this is happening. Ideally it should be a tensor of shape (2,1,28,28).
EDIT 2:
Inside `prepare_train_features`, the shape of `images[0]` is `torch.Size([1,28,28])`, the conversion is working. However, the output of the `map` is a list of list of list of list.
Okay, that makes sense.
Raw images are list of Array2D, hence we get a single tensor when `set_format` is used. But, why should I need to convert the raw images to `torch` format when `map` does this internally?
Using `Array3D` did not work with `map` when raw images weren't `set_format`ted to torch type. | [
-0.1273753047,
-0.3511653543,
-0.0179326311,
0.3536090851,
0.4760026336,
0.0887137353,
0.7330292463,
0.3804347813,
0.0655257925,
-0.0380426571,
-0.1111534089,
0.3819510639,
-0.2329690456,
-0.3339343965,
0.0351092033,
-0.56149441,
0.2225798368,
-0.0998275802,
-0.2807980776,
-0.0613473542,
-0.2431338727,
0.0354893208,
-0.2184972465,
-0.1084965691,
-0.5479356647,
0.0440960862,
-0.0195555668,
-0.0765686631,
-0.0622991286,
-0.1391801089,
0.1223466247,
-0.1744965017,
0.4444538355,
0.7012932897,
-0.0001277011,
0.0703017637,
0.3642728031,
-0.1737782061,
0.0499993414,
-0.161013633,
0.0400471762,
-0.2573826909,
0.072776489,
-0.0946833491,
-0.2234944403,
-0.1708453,
0.0959102288,
-0.1790296137,
-0.0281420499,
0.4191690087,
0.0771299899,
0.2790107131,
0.2466612458,
0.1386306435,
0.22781156,
0.5182866454,
-0.273437947,
-0.056117028,
0.2261502147,
0.3754776716,
0.1240048036,
0.5647348166,
-0.2913349569,
-0.0362339392,
0.2669452727,
0.1290050745,
-0.1014554128,
-0.3140053451,
0.0491572432,
0.0894629955,
0.4219443202,
-0.1494392157,
-0.0807788968,
-0.1436638385,
-0.1439783871,
-0.1273806691,
0.040591076,
0.2803193629,
-0.0718411431,
-0.1872384697,
-0.5392424464,
0.3052917719,
-0.1486236155,
0.2131593376,
-0.2805242538,
-0.0034206749,
-0.1381632388,
0.2928087413,
-0.0471191294,
-0.1127126515,
0.193081066,
-0.1234149858,
0.1300754547,
-0.0098257521,
-0.14363195,
-0.0534906499,
-0.3895993531,
-0.3150125742,
-0.2001478821,
-0.1602652222,
0.1664854437,
0.1392757148,
-0.1163955703,
0.1280710101,
0.182633087,
0.2680341601,
0.105782561,
0.3207030296,
-0.0858925655,
-0.2895815372,
-0.0469193235,
0.0877334625,
-0.2962266505,
-0.4357524216,
0.1545345932,
0.3252967596,
0.245221287,
0.0687427744,
0.1298556328,
-0.3239411414,
-0.1089226305,
-0.009586527,
0.0752253011,
0.2850383818,
-0.118679136,
0.2439045608,
0.4804832935,
0.397203058,
-0.1538182348,
0.0695628896,
0.0250724126,
0.0315210149,
-0.4404955208,
-0.2856985331,
0.1534095705,
-0.1177731901,
0.1000702605,
0.0374316536,
0.0464156754,
0.1006964073,
0.073496595,
-0.1858698726,
0.6246293187,
0.1476473957,
-0.1944741011,
0.2767930329,
0.2709067166,
0.5297800303,
-0.3042984605,
0.2565315962,
-0.5095698833,
0.065252535,
-0.0230915733,
-0.0145439208,
0.218655169,
-0.0207218453,
-0.21216245,
0.0158290844,
0.5923122764,
-0.0836781189,
0.2734875381,
-0.5791625381,
0.0949641094,
-0.1913349926,
0.0341524445,
0.0636693314,
-0.2047153264,
-0.0523584448,
0.3863435686,
0.091949828,
0.3154586852,
0.1602820307,
0.0510832109,
0.0798702538,
-0.2253529727,
-0.2350506932,
0.3363234401,
-0.3768563569,
0.0920385197,
-0.0176934246,
0.0938790515,
-0.0638039783,
0.1120189652,
0.3361780643,
0.0326006748,
-0.1280014962,
0.0294486172,
0.1963144988,
-0.1661821902,
0.1402870268,
0.0773596242,
0.1913028955,
0.1933744848,
0.0350064598,
-0.0156837292,
0.2076538354,
-0.2216716707,
0.1775766164,
-0.0542802736,
-0.0747132748,
0.0711774975,
0.0403781086,
-0.3698906004,
-0.0121967876,
-0.0474697649,
0.1864667982,
-0.2331001908,
-0.0487212278,
0.023635203,
0.0822170898,
0.0582790896,
0.0479247868,
0.0049779508,
-0.1919566393,
-0.1822059304,
-0.1390273869,
-0.0032421914,
0.0922106206,
-0.2094768882,
-0.261724323,
-0.0523434915,
-0.1232490093,
0.1783040464,
0.0588377379,
-0.3590368927,
0.0510256998,
0.0192736685,
-0.3653306365,
-0.2013597339,
0.1733922958,
0.2676690817,
-0.0996205956,
-0.042234946,
0.1916034222,
0.0632891431,
0.1146329641,
-0.5482743979,
0.0834657177,
0.1778421402,
-0.2469699979,
0.2043909729,
0.21627976,
0.148533985,
-0.2755034566,
-0.1960351765,
0.4387867153,
0.1604105979,
0.3159126043,
-0.3300259113,
0.1161018088,
-0.0726466849,
-0.0394020006,
-0.1906338334,
-0.0408079401,
-0.2772819102,
0.0730236918,
-0.1318594962,
0.0129819224,
-0.456918478,
-0.0449361987,
0.2962616384,
0.0887638927,
0.1758723557,
-0.0012161626,
-0.3998460472,
0.2133122683,
0.1771266311,
-0.6013534069,
0.2978382111,
-0.0043618386,
0.1892073452,
-0.1050186977,
0.095583491,
-0.1625432819,
0.2484446168,
0.1140421703,
0.0907485485,
-0.1994648427,
0.1418524981,
-0.1079259813,
0.0268464927,
0.0263705309,
-0.1406288743,
-0.2336928695,
-0.3589506149,
0.2713824809,
-0.1661831439,
-0.1345184743,
-0.4018547535,
-0.2621857822,
0.0360562503,
-0.0740049183,
-0.082333751,
0.1507584006,
0.1855680048,
0.1904348731,
0.2634178698,
-0.1818944663,
0.3743163645,
-0.4130294323,
-0.035354875,
-0.0750098228,
-0.286796093,
-0.0991648808,
0.0896559656,
-0.5234106183,
0.0914844051,
-0.2289900333,
-0.0931909382,
-0.2501759827,
0.0135479644,
0.1418222338,
-0.2678501606,
-0.2850028276,
0.3085912764,
0.1939282864,
-0.1424821913,
0.0406621695,
0.2663511932,
0.0103071555,
0.0469012447,
0.1537835598,
-0.0896200985,
0.1165603474,
-0.0632982552,
-0.1767407805,
-0.0966179743,
-0.075061664,
-0.070416607,
-0.0217598137,
0.0633770078,
0.3462583721,
0.372183919,
-0.0898906365,
-0.0520421751,
0.0356090553,
0.0629648119,
-0.3976877034,
0.5225031972,
-0.2261542529,
-0.3608260751,
0.1192018762,
0.0238558389,
0.0651470646,
0.4862686098,
0.060522791,
0.0497318283,
0.0117822066,
-0.0789449736,
0.2525925934,
0.2211973071,
0.3510199785,
-0.0911250561,
0.0191657748,
-0.1647070944,
0.0154164825,
-0.1018714905,
-0.0632553846,
0.6088866591,
0.1850842088,
0.2399428785,
0.0992584452,
0.6996648312,
-0.0611371584,
-0.7380948067,
0.2168068886,
-0.242509678,
0.1921667606,
-0.1181218103,
-0.3539583385,
0.3988562822,
-0.2094198763,
0.0643741786,
-0.0859674439,
-0.024907032,
-0.2391579151,
-0.0902244151,
0.3573616743,
-0.3172068596,
-0.1585739851,
0.4044299424,
-0.1447023898,
0.2763730288,
-0.2313425988,
0.3245900273,
-0.2609262168,
-0.2137925476,
0.0600569248,
0.4487719536,
0.1385134459,
0.0417248979,
-0.3342843056,
-0.2166966051,
-0.1432270408,
0.3568275571,
0.1195773706,
0.5672698021,
-0.0980362967,
-0.0744651258,
0.1015565023,
0.2413697243,
0.8307590485,
0.0455573462,
-0.0904591605,
0.1514422297,
-0.3478347957,
-0.4549783468,
-0.1022293717,
-0.0514177196,
0.0906814784,
0.171690315,
0.1203863472,
-0.0910536647,
-0.1833340377,
-0.0058328966,
0.1955551356,
-0.0551247038,
0.0347188041,
-0.2269114703,
0.058757659,
-0.1296496391,
-0.1552832723,
0.2211825401,
0.0296756551,
0.0051617255,
-0.3081283569,
-0.3262304068,
-0.1266996413,
0.1824819148,
-0.1330122203,
0.4110674262,
0.0992513523,
-0.1780855954,
0.076906763,
0.5561057329,
0.4184041321,
-0.0389392413,
-0.1187734976,
0.0806434527,
0.2931110561,
0.1064323112,
0.3002042174,
0.320822835,
-0.0618730187,
-0.4396643341,
-0.2268486619,
-0.1938148439,
-0.3305326402,
0.3972931504,
0.4080632627,
0.0989354551,
-0.4953554273,
-0.4921705425,
0.3861900568,
0.2937192619,
0.1958300769,
0.2045917213,
-0.5417593122,
-0.4009674489,
0.0781785697,
0.4662418664,
0.8556217551,
-0.037078727,
0.3702827692,
0.0315655693,
0.0108071193,
0.1721023768,
-0.0018886278,
0.4117948115,
-0.3542813957,
0.1392895281,
-0.1648537517,
-0.3125495315,
0.2525228262,
-0.060203135,
-0.2439696491,
0.0425363369,
-0.3494715691,
0.1936195642,
-0.1256936193,
-0.0828530714,
-0.0498264618,
-0.4714174271,
0.1878736466,
0.0154520553,
-0.0825134888,
0.2617389858,
0.1111611798,
-0.1636207998,
-0.3798605204,
0.0616962239,
-0.0762221143,
-0.0150117185,
-0.2459690571,
-0.1632432491,
-0.0243102051,
-0.7298656702,
0.0586798526,
0.4961580336,
0.3345546722,
-0.160566479,
-0.0478368364,
0.1396413594,
0.4438471496,
0.1629004925,
-0.0990172699,
-0.3401843011,
0.2853403986,
0.0973753631,
0.1843320727,
0.1086379662,
0.0681485757,
0.2105205804,
-0.577789247,
0.0372510366,
0.3060290217,
-0.3449506164,
-0.3229845464,
-0.1984502971,
0.0491850227,
0.0381633341,
0.0286184791,
0.124488771,
-0.0389032736,
0.2072432339,
-0.0415120944,
-0.2264618576,
0.1328899711,
0.4523314238,
0.112983726,
-0.3500464261,
0.3907569349,
0.3549591601,
-0.0447779372,
-0.0437441096,
0.4166942239,
0.6353191733,
-0.3456966281,
0.0994976684,
-0.1027166471,
-0.1569870114,
-0.0110735875,
0.5430940986,
-0.2343174964,
-0.1309778541,
-0.2389573902,
-0.0408173315,
-0.4308937788,
0.0108135957,
-0.3162982762,
0.456630528,
-0.0432226621,
0.3627102971,
0.210505724,
-0.1873493046,
-0.1441360116,
-0.2586487532,
0.1723508388,
0.3679335117,
-0.2471824437,
0.2187612951,
-0.1269584745,
-0.1220230311,
-0.0362523533,
0.0260314047,
-0.2497397214,
-0.0333907679,
-0.0576779619,
0.1773060858,
0.0666143522,
-0.1135402918,
-0.0735467896,
-0.1736695915,
-0.1385810077,
-0.2681719065,
-0.037718121,
0.1109212413,
-0.11599949,
0.3531612456,
-0.178366214,
0.2312256396,
0.3186765909,
-0.2700001597,
-0.1026224792,
0.0358713232,
0.2229627371,
0.0600504763,
0.0045319153,
-0.0615568496,
-0.2772366107,
0.1125916764,
0.1652028263,
0.2549746037,
0.2046174854,
-0.0999964103,
0.1091783047,
-0.0438066572,
0.2041842341,
0.1012554616,
-0.1718705595,
-0.050828699,
0.0603729896,
-0.0076234438,
0.1006219834,
0.1449991316,
0.0388691351,
0.1252815872,
-0.0834641606,
0.275578022,
0.0665585697,
0.3113947809,
-0.3705078661,
0.0451425537,
0.4178565443,
-0.2410733104,
0.2350862026,
0.4640147686,
0.0147997756,
0.1930496842,
0.290704608,
0.1093435511,
-0.1187017635,
0.3226963878,
0.3945167661,
0.820430994,
0.5895959139,
0.0837696865,
0.2746027708,
0.0992569551,
-0.1536075622,
-0.2072515339,
0.2358236462,
0.3919682801,
0.0188666489,
0.1856931895,
-0.2407990843,
-0.4871240854,
0.225882113,
0.1217747778,
-0.1946178228,
-0.082223542,
-0.6648060083,
0.0118752681,
0.1358262002,
-0.1866595894,
-0.1797155738,
-0.0501377247,
0.2205908448,
0.2597535551,
-0.1032206789,
-0.1609123051,
-0.1724743992,
0.0691012219,
0.037529368,
-0.007329775,
0.1697572321,
-0.1368460655,
0.1617608517,
0.2448244244,
0.1969081759,
0.284953326,
0.4870801568,
-0.1681717485,
-0.1598939151,
-0.0105530387,
-0.1923659146,
0.0899016932,
0.066711925,
0.031533774,
-0.1532076597,
-0.0149563281,
0.0084625762,
0.0386277921,
0.0314605534,
-0.0940808728,
-0.2182596475,
0.1676249504,
0.3055749238,
-0.3637773693,
-0.1490927637,
-0.0998983905,
-0.2466560602,
-0.3483100832,
-0.2655346692,
0.5327388048,
0.0278958473,
0.1280099303,
0.1421680599,
-0.0421595387,
0.0487859175,
0.1574119478,
-0.121556811,
0.0775766447,
-0.1540937126,
0.1359660923,
-0.0858048201,
0.0319104381,
0.1184747368,
-0.3834479749,
-0.0188493319,
-0.0391645581,
0.2425420135,
0.0646747723,
0.2020902038,
-0.0983472839,
0.4987450838,
0.6146567464,
-0.1336154193,
-0.1414809078,
-0.1304193139,
0.3706512451,
0.0733085498,
-0.0774656981,
0.0219215602,
0.1024274155,
0.0065069334,
-0.3420648277,
-0.2554903924,
-0.0199823231,
0.3041450083,
0.3512826264,
0.0932883546,
0.3203133047,
-0.0192206632,
0.0484100245,
-0.2411341518,
0.0504153185,
-0.1276014,
0.0096705668,
-0.0228027813,
0.5810170174,
-0.224402234,
-0.059342429,
-0.4136501551,
0.4290263653,
0.0905311108,
-0.3388552368,
-0.0805192441,
0.3583999276,
-0.3173208535,
-0.2010883093,
0.226195246,
0.410022229,
0.1513598859,
0.1943574995,
-0.5254407525,
-0.4069116116,
0.4472022951,
-0.4417079389,
-0.5069123507,
0.0290744491,
-0.3133937716,
0.0679039732,
0.4964230061,
-0.4710497558,
-0.1491341293,
0.0015052542,
-0.080827713,
-0.0916047096,
-0.0981937125,
0.2610401213,
0.0151107861,
-0.2518463135,
0.1790072173,
0.1764502972,
-0.0228159856,
-0.4204159677,
-0.2753599882
]
|
https://github.com/huggingface/datasets/issues/2005 | Setting to torch format not working with torchvision and MNIST | I understand that `map` needs to know what kind of output tensors are expected, and thus converting the raw dataset to `torch` format is necessary. Closing the issue since it is resolved. | Hi
I am trying to use `torchvision.transforms` to handle the transformation of the image data in the `mnist` dataset. Assume I have a `transform` variable which contains the `torchvision.transforms` object.
A snippet of what I am trying to do:
```python
def prepare_features(examples):
images = []
labels = []
for example_idx, example in enumerate(examples["image"]):
if transform is not None:
images.append(transform(
np.array(examples["image"][example_idx], dtype=np.uint8)
))
else:
images.append(torch.tensor(np.array(examples["image"][example_idx], dtype=np.uint8)))
labels.append(torch.tensor(examples["label"][example_idx]))
output = {"label":labels, "image":images}
return output
raw_dataset = load_dataset('mnist')
train_dataset = raw_dataset.map(prepare_features, batched=True, batch_size=10000)
train_dataset.set_format("torch",columns=["image","label"])
```
After this, I check the type of the following:
```python
print(type(train_dataset["train"]["label"]))
print(type(train_dataset["train"]["image"][0]))
```
This leads to the following output:
```python
<class 'torch.Tensor'>
<class 'list'>
```
I use `torch.utils.DataLoader` for batches, the type of `batch["train"]["image"]` is also `<class 'list'>`.
I don't understand why only the `label` is converted to a torch tensor, why does the image not get converted? How can I fix this issue?
Thanks,
Gunjan
EDIT:
I just checked the shapes, and the types, `batch[image]` is a actually a list of list of tensors. Shape is (1,28,2,28), where `batch_size` is 2. I don't understand why this is happening. Ideally it should be a tensor of shape (2,1,28,28).
EDIT 2:
Inside `prepare_train_features`, the shape of `images[0]` is `torch.Size([1,28,28])`, the conversion is working. However, the output of the `map` is a list of list of list of list. | 32 | Setting to torch format not working with torchvision and MNIST
Hi
I am trying to use `torchvision.transforms` to handle the transformation of the image data in the `mnist` dataset. Assume I have a `transform` variable which contains the `torchvision.transforms` object.
A snippet of what I am trying to do:
```python
def prepare_features(examples):
images = []
labels = []
for example_idx, example in enumerate(examples["image"]):
if transform is not None:
images.append(transform(
np.array(examples["image"][example_idx], dtype=np.uint8)
))
else:
images.append(torch.tensor(np.array(examples["image"][example_idx], dtype=np.uint8)))
labels.append(torch.tensor(examples["label"][example_idx]))
output = {"label":labels, "image":images}
return output
raw_dataset = load_dataset('mnist')
train_dataset = raw_dataset.map(prepare_features, batched=True, batch_size=10000)
train_dataset.set_format("torch",columns=["image","label"])
```
After this, I check the type of the following:
```python
print(type(train_dataset["train"]["label"]))
print(type(train_dataset["train"]["image"][0]))
```
This leads to the following output:
```python
<class 'torch.Tensor'>
<class 'list'>
```
I use `torch.utils.DataLoader` for batches, the type of `batch["train"]["image"]` is also `<class 'list'>`.
I don't understand why only the `label` is converted to a torch tensor, why does the image not get converted? How can I fix this issue?
Thanks,
Gunjan
EDIT:
I just checked the shapes, and the types, `batch[image]` is a actually a list of list of tensors. Shape is (1,28,2,28), where `batch_size` is 2. I don't understand why this is happening. Ideally it should be a tensor of shape (2,1,28,28).
EDIT 2:
Inside `prepare_train_features`, the shape of `images[0]` is `torch.Size([1,28,28])`, the conversion is working. However, the output of the `map` is a list of list of list of list.
I understand that `map` needs to know what kind of output tensors are expected, and thus converting the raw dataset to `torch` format is necessary. Closing the issue since it is resolved. | [
-0.1273753047,
-0.3511653543,
-0.0179326311,
0.3536090851,
0.4760026336,
0.0887137353,
0.7330292463,
0.3804347813,
0.0655257925,
-0.0380426571,
-0.1111534089,
0.3819510639,
-0.2329690456,
-0.3339343965,
0.0351092033,
-0.56149441,
0.2225798368,
-0.0998275802,
-0.2807980776,
-0.0613473542,
-0.2431338727,
0.0354893208,
-0.2184972465,
-0.1084965691,
-0.5479356647,
0.0440960862,
-0.0195555668,
-0.0765686631,
-0.0622991286,
-0.1391801089,
0.1223466247,
-0.1744965017,
0.4444538355,
0.7012932897,
-0.0001277011,
0.0703017637,
0.3642728031,
-0.1737782061,
0.0499993414,
-0.161013633,
0.0400471762,
-0.2573826909,
0.072776489,
-0.0946833491,
-0.2234944403,
-0.1708453,
0.0959102288,
-0.1790296137,
-0.0281420499,
0.4191690087,
0.0771299899,
0.2790107131,
0.2466612458,
0.1386306435,
0.22781156,
0.5182866454,
-0.273437947,
-0.056117028,
0.2261502147,
0.3754776716,
0.1240048036,
0.5647348166,
-0.2913349569,
-0.0362339392,
0.2669452727,
0.1290050745,
-0.1014554128,
-0.3140053451,
0.0491572432,
0.0894629955,
0.4219443202,
-0.1494392157,
-0.0807788968,
-0.1436638385,
-0.1439783871,
-0.1273806691,
0.040591076,
0.2803193629,
-0.0718411431,
-0.1872384697,
-0.5392424464,
0.3052917719,
-0.1486236155,
0.2131593376,
-0.2805242538,
-0.0034206749,
-0.1381632388,
0.2928087413,
-0.0471191294,
-0.1127126515,
0.193081066,
-0.1234149858,
0.1300754547,
-0.0098257521,
-0.14363195,
-0.0534906499,
-0.3895993531,
-0.3150125742,
-0.2001478821,
-0.1602652222,
0.1664854437,
0.1392757148,
-0.1163955703,
0.1280710101,
0.182633087,
0.2680341601,
0.105782561,
0.3207030296,
-0.0858925655,
-0.2895815372,
-0.0469193235,
0.0877334625,
-0.2962266505,
-0.4357524216,
0.1545345932,
0.3252967596,
0.245221287,
0.0687427744,
0.1298556328,
-0.3239411414,
-0.1089226305,
-0.009586527,
0.0752253011,
0.2850383818,
-0.118679136,
0.2439045608,
0.4804832935,
0.397203058,
-0.1538182348,
0.0695628896,
0.0250724126,
0.0315210149,
-0.4404955208,
-0.2856985331,
0.1534095705,
-0.1177731901,
0.1000702605,
0.0374316536,
0.0464156754,
0.1006964073,
0.073496595,
-0.1858698726,
0.6246293187,
0.1476473957,
-0.1944741011,
0.2767930329,
0.2709067166,
0.5297800303,
-0.3042984605,
0.2565315962,
-0.5095698833,
0.065252535,
-0.0230915733,
-0.0145439208,
0.218655169,
-0.0207218453,
-0.21216245,
0.0158290844,
0.5923122764,
-0.0836781189,
0.2734875381,
-0.5791625381,
0.0949641094,
-0.1913349926,
0.0341524445,
0.0636693314,
-0.2047153264,
-0.0523584448,
0.3863435686,
0.091949828,
0.3154586852,
0.1602820307,
0.0510832109,
0.0798702538,
-0.2253529727,
-0.2350506932,
0.3363234401,
-0.3768563569,
0.0920385197,
-0.0176934246,
0.0938790515,
-0.0638039783,
0.1120189652,
0.3361780643,
0.0326006748,
-0.1280014962,
0.0294486172,
0.1963144988,
-0.1661821902,
0.1402870268,
0.0773596242,
0.1913028955,
0.1933744848,
0.0350064598,
-0.0156837292,
0.2076538354,
-0.2216716707,
0.1775766164,
-0.0542802736,
-0.0747132748,
0.0711774975,
0.0403781086,
-0.3698906004,
-0.0121967876,
-0.0474697649,
0.1864667982,
-0.2331001908,
-0.0487212278,
0.023635203,
0.0822170898,
0.0582790896,
0.0479247868,
0.0049779508,
-0.1919566393,
-0.1822059304,
-0.1390273869,
-0.0032421914,
0.0922106206,
-0.2094768882,
-0.261724323,
-0.0523434915,
-0.1232490093,
0.1783040464,
0.0588377379,
-0.3590368927,
0.0510256998,
0.0192736685,
-0.3653306365,
-0.2013597339,
0.1733922958,
0.2676690817,
-0.0996205956,
-0.042234946,
0.1916034222,
0.0632891431,
0.1146329641,
-0.5482743979,
0.0834657177,
0.1778421402,
-0.2469699979,
0.2043909729,
0.21627976,
0.148533985,
-0.2755034566,
-0.1960351765,
0.4387867153,
0.1604105979,
0.3159126043,
-0.3300259113,
0.1161018088,
-0.0726466849,
-0.0394020006,
-0.1906338334,
-0.0408079401,
-0.2772819102,
0.0730236918,
-0.1318594962,
0.0129819224,
-0.456918478,
-0.0449361987,
0.2962616384,
0.0887638927,
0.1758723557,
-0.0012161626,
-0.3998460472,
0.2133122683,
0.1771266311,
-0.6013534069,
0.2978382111,
-0.0043618386,
0.1892073452,
-0.1050186977,
0.095583491,
-0.1625432819,
0.2484446168,
0.1140421703,
0.0907485485,
-0.1994648427,
0.1418524981,
-0.1079259813,
0.0268464927,
0.0263705309,
-0.1406288743,
-0.2336928695,
-0.3589506149,
0.2713824809,
-0.1661831439,
-0.1345184743,
-0.4018547535,
-0.2621857822,
0.0360562503,
-0.0740049183,
-0.082333751,
0.1507584006,
0.1855680048,
0.1904348731,
0.2634178698,
-0.1818944663,
0.3743163645,
-0.4130294323,
-0.035354875,
-0.0750098228,
-0.286796093,
-0.0991648808,
0.0896559656,
-0.5234106183,
0.0914844051,
-0.2289900333,
-0.0931909382,
-0.2501759827,
0.0135479644,
0.1418222338,
-0.2678501606,
-0.2850028276,
0.3085912764,
0.1939282864,
-0.1424821913,
0.0406621695,
0.2663511932,
0.0103071555,
0.0469012447,
0.1537835598,
-0.0896200985,
0.1165603474,
-0.0632982552,
-0.1767407805,
-0.0966179743,
-0.075061664,
-0.070416607,
-0.0217598137,
0.0633770078,
0.3462583721,
0.372183919,
-0.0898906365,
-0.0520421751,
0.0356090553,
0.0629648119,
-0.3976877034,
0.5225031972,
-0.2261542529,
-0.3608260751,
0.1192018762,
0.0238558389,
0.0651470646,
0.4862686098,
0.060522791,
0.0497318283,
0.0117822066,
-0.0789449736,
0.2525925934,
0.2211973071,
0.3510199785,
-0.0911250561,
0.0191657748,
-0.1647070944,
0.0154164825,
-0.1018714905,
-0.0632553846,
0.6088866591,
0.1850842088,
0.2399428785,
0.0992584452,
0.6996648312,
-0.0611371584,
-0.7380948067,
0.2168068886,
-0.242509678,
0.1921667606,
-0.1181218103,
-0.3539583385,
0.3988562822,
-0.2094198763,
0.0643741786,
-0.0859674439,
-0.024907032,
-0.2391579151,
-0.0902244151,
0.3573616743,
-0.3172068596,
-0.1585739851,
0.4044299424,
-0.1447023898,
0.2763730288,
-0.2313425988,
0.3245900273,
-0.2609262168,
-0.2137925476,
0.0600569248,
0.4487719536,
0.1385134459,
0.0417248979,
-0.3342843056,
-0.2166966051,
-0.1432270408,
0.3568275571,
0.1195773706,
0.5672698021,
-0.0980362967,
-0.0744651258,
0.1015565023,
0.2413697243,
0.8307590485,
0.0455573462,
-0.0904591605,
0.1514422297,
-0.3478347957,
-0.4549783468,
-0.1022293717,
-0.0514177196,
0.0906814784,
0.171690315,
0.1203863472,
-0.0910536647,
-0.1833340377,
-0.0058328966,
0.1955551356,
-0.0551247038,
0.0347188041,
-0.2269114703,
0.058757659,
-0.1296496391,
-0.1552832723,
0.2211825401,
0.0296756551,
0.0051617255,
-0.3081283569,
-0.3262304068,
-0.1266996413,
0.1824819148,
-0.1330122203,
0.4110674262,
0.0992513523,
-0.1780855954,
0.076906763,
0.5561057329,
0.4184041321,
-0.0389392413,
-0.1187734976,
0.0806434527,
0.2931110561,
0.1064323112,
0.3002042174,
0.320822835,
-0.0618730187,
-0.4396643341,
-0.2268486619,
-0.1938148439,
-0.3305326402,
0.3972931504,
0.4080632627,
0.0989354551,
-0.4953554273,
-0.4921705425,
0.3861900568,
0.2937192619,
0.1958300769,
0.2045917213,
-0.5417593122,
-0.4009674489,
0.0781785697,
0.4662418664,
0.8556217551,
-0.037078727,
0.3702827692,
0.0315655693,
0.0108071193,
0.1721023768,
-0.0018886278,
0.4117948115,
-0.3542813957,
0.1392895281,
-0.1648537517,
-0.3125495315,
0.2525228262,
-0.060203135,
-0.2439696491,
0.0425363369,
-0.3494715691,
0.1936195642,
-0.1256936193,
-0.0828530714,
-0.0498264618,
-0.4714174271,
0.1878736466,
0.0154520553,
-0.0825134888,
0.2617389858,
0.1111611798,
-0.1636207998,
-0.3798605204,
0.0616962239,
-0.0762221143,
-0.0150117185,
-0.2459690571,
-0.1632432491,
-0.0243102051,
-0.7298656702,
0.0586798526,
0.4961580336,
0.3345546722,
-0.160566479,
-0.0478368364,
0.1396413594,
0.4438471496,
0.1629004925,
-0.0990172699,
-0.3401843011,
0.2853403986,
0.0973753631,
0.1843320727,
0.1086379662,
0.0681485757,
0.2105205804,
-0.577789247,
0.0372510366,
0.3060290217,
-0.3449506164,
-0.3229845464,
-0.1984502971,
0.0491850227,
0.0381633341,
0.0286184791,
0.124488771,
-0.0389032736,
0.2072432339,
-0.0415120944,
-0.2264618576,
0.1328899711,
0.4523314238,
0.112983726,
-0.3500464261,
0.3907569349,
0.3549591601,
-0.0447779372,
-0.0437441096,
0.4166942239,
0.6353191733,
-0.3456966281,
0.0994976684,
-0.1027166471,
-0.1569870114,
-0.0110735875,
0.5430940986,
-0.2343174964,
-0.1309778541,
-0.2389573902,
-0.0408173315,
-0.4308937788,
0.0108135957,
-0.3162982762,
0.456630528,
-0.0432226621,
0.3627102971,
0.210505724,
-0.1873493046,
-0.1441360116,
-0.2586487532,
0.1723508388,
0.3679335117,
-0.2471824437,
0.2187612951,
-0.1269584745,
-0.1220230311,
-0.0362523533,
0.0260314047,
-0.2497397214,
-0.0333907679,
-0.0576779619,
0.1773060858,
0.0666143522,
-0.1135402918,
-0.0735467896,
-0.1736695915,
-0.1385810077,
-0.2681719065,
-0.037718121,
0.1109212413,
-0.11599949,
0.3531612456,
-0.178366214,
0.2312256396,
0.3186765909,
-0.2700001597,
-0.1026224792,
0.0358713232,
0.2229627371,
0.0600504763,
0.0045319153,
-0.0615568496,
-0.2772366107,
0.1125916764,
0.1652028263,
0.2549746037,
0.2046174854,
-0.0999964103,
0.1091783047,
-0.0438066572,
0.2041842341,
0.1012554616,
-0.1718705595,
-0.050828699,
0.0603729896,
-0.0076234438,
0.1006219834,
0.1449991316,
0.0388691351,
0.1252815872,
-0.0834641606,
0.275578022,
0.0665585697,
0.3113947809,
-0.3705078661,
0.0451425537,
0.4178565443,
-0.2410733104,
0.2350862026,
0.4640147686,
0.0147997756,
0.1930496842,
0.290704608,
0.1093435511,
-0.1187017635,
0.3226963878,
0.3945167661,
0.820430994,
0.5895959139,
0.0837696865,
0.2746027708,
0.0992569551,
-0.1536075622,
-0.2072515339,
0.2358236462,
0.3919682801,
0.0188666489,
0.1856931895,
-0.2407990843,
-0.4871240854,
0.225882113,
0.1217747778,
-0.1946178228,
-0.082223542,
-0.6648060083,
0.0118752681,
0.1358262002,
-0.1866595894,
-0.1797155738,
-0.0501377247,
0.2205908448,
0.2597535551,
-0.1032206789,
-0.1609123051,
-0.1724743992,
0.0691012219,
0.037529368,
-0.007329775,
0.1697572321,
-0.1368460655,
0.1617608517,
0.2448244244,
0.1969081759,
0.284953326,
0.4870801568,
-0.1681717485,
-0.1598939151,
-0.0105530387,
-0.1923659146,
0.0899016932,
0.066711925,
0.031533774,
-0.1532076597,
-0.0149563281,
0.0084625762,
0.0386277921,
0.0314605534,
-0.0940808728,
-0.2182596475,
0.1676249504,
0.3055749238,
-0.3637773693,
-0.1490927637,
-0.0998983905,
-0.2466560602,
-0.3483100832,
-0.2655346692,
0.5327388048,
0.0278958473,
0.1280099303,
0.1421680599,
-0.0421595387,
0.0487859175,
0.1574119478,
-0.121556811,
0.0775766447,
-0.1540937126,
0.1359660923,
-0.0858048201,
0.0319104381,
0.1184747368,
-0.3834479749,
-0.0188493319,
-0.0391645581,
0.2425420135,
0.0646747723,
0.2020902038,
-0.0983472839,
0.4987450838,
0.6146567464,
-0.1336154193,
-0.1414809078,
-0.1304193139,
0.3706512451,
0.0733085498,
-0.0774656981,
0.0219215602,
0.1024274155,
0.0065069334,
-0.3420648277,
-0.2554903924,
-0.0199823231,
0.3041450083,
0.3512826264,
0.0932883546,
0.3203133047,
-0.0192206632,
0.0484100245,
-0.2411341518,
0.0504153185,
-0.1276014,
0.0096705668,
-0.0228027813,
0.5810170174,
-0.224402234,
-0.059342429,
-0.4136501551,
0.4290263653,
0.0905311108,
-0.3388552368,
-0.0805192441,
0.3583999276,
-0.3173208535,
-0.2010883093,
0.226195246,
0.410022229,
0.1513598859,
0.1943574995,
-0.5254407525,
-0.4069116116,
0.4472022951,
-0.4417079389,
-0.5069123507,
0.0290744491,
-0.3133937716,
0.0679039732,
0.4964230061,
-0.4710497558,
-0.1491341293,
0.0015052542,
-0.080827713,
-0.0916047096,
-0.0981937125,
0.2610401213,
0.0151107861,
-0.2518463135,
0.1790072173,
0.1764502972,
-0.0228159856,
-0.4204159677,
-0.2753599882
]
|
https://github.com/huggingface/datasets/issues/2003 | Messages are being printed to the `stdout` | This is expected to show this message to the user via stdout.
This way the users see it directly and can cancel the downloading if they want to.
Could you elaborate why it would be better to have it in stderr instead of stdout ? | In this code segment, we can see some messages are being printed to the `stdout`.
https://github.com/huggingface/datasets/blob/7e60bb509b595e8edc60a87f32b2bacfc065d607/src/datasets/builder.py#L545-L554
According to the comment, it is done intentionally, but I don't really understand why don't we log it with a higher level or print it directly to the `stderr`.
In my opinion, this kind of messages should never printed to the stdout. At least some configuration/flag should make it possible to provide in order to explicitly prevent the package to contaminate the stdout.
| 45 | Messages are being printed to the `stdout`
In this code segment, we can see some messages are being printed to the `stdout`.
https://github.com/huggingface/datasets/blob/7e60bb509b595e8edc60a87f32b2bacfc065d607/src/datasets/builder.py#L545-L554
According to the comment, it is done intentionally, but I don't really understand why don't we log it with a higher level or print it directly to the `stderr`.
In my opinion, this kind of messages should never printed to the stdout. At least some configuration/flag should make it possible to provide in order to explicitly prevent the package to contaminate the stdout.
This is expected to show this message to the user via stdout.
This way the users see it directly and can cancel the downloading if they want to.
Could you elaborate why it would be better to have it in stderr instead of stdout ? | [
-0.0470960625,
-0.3709275424,
-0.0396359488,
0.270612061,
0.2318518311,
-0.0572963841,
0.2466088235,
0.1149494946,
-0.0417822339,
0.1984181851,
0.1732619703,
0.1642703563,
-0.1199179217,
0.368881762,
0.1807005703,
0.1382276416,
-0.0852123499,
-0.1296654493,
-0.3972430825,
0.2983495295,
0.1317114979,
0.1694650352,
0.3729339838,
0.4860754013,
-0.5674238801,
-0.0275850762,
0.2408997566,
-0.0820058361,
-0.2319761217,
-0.5787137151,
0.0741454363,
0.1343486011,
0.1837683469,
0.1799419224,
-0.0001180599,
0.144953087,
0.4684005976,
0.2060817629,
-0.6819634438,
-0.0856235027,
-0.1658634245,
-0.1279905289,
0.3208130896,
-0.0690777451,
0.1317874342,
-0.2993817329,
0.2840439677,
-0.3241887689,
0.1400138587,
0.4661121666,
0.1768934131,
0.2232481837,
0.0355277248,
0.2963495851,
0.2246191204,
0.3057036102,
-0.064274393,
0.0225169845,
0.2490638345,
0.5164732933,
-0.0466827787,
0.3044887781,
-0.1097129211,
-0.3461034298,
0.1512935758,
0.183387816,
0.1994552314,
-0.2152293175,
0.1340061724,
0.0134586059,
0.5597183108,
-0.4376441836,
-0.1601392478,
-0.2399808913,
-0.1339332014,
-0.1432036906,
0.229441002,
0.1206061766,
-0.2808731794,
0.499940455,
-0.3562499583,
-0.3182742298,
-0.2685394585,
-0.0708174631,
-0.0102503225,
-0.1528874934,
-0.2506072223,
0.1657453626,
-0.1827572286,
0.2157797068,
-0.0832351819,
-0.2934995592,
-0.0698479638,
-0.1787753105,
0.0691014603,
-0.0906247571,
-0.0050331135,
-0.1460137665,
-0.0950105786,
-0.028815385,
-0.002384593,
-0.0100430045,
0.3453406096,
0.0766511485,
0.4637839496,
0.0726138949,
0.3933345973,
0.1709567159,
-0.0247005634,
0.1267796755,
0.3316876888,
-0.3063759208,
0.3164299726,
0.2413711846,
0.1670805067,
-0.3005856276,
0.1296256036,
-0.3068225086,
-0.0729349032,
0.0900440291,
-0.0182395224,
0.0932417661,
0.0036977883,
0.1688581556,
0.1365754604,
-0.0851945207,
0.1672448069,
0.027933592,
0.2186057717,
0.0639154091,
-0.1007549986,
0.0687320158,
-0.1920706779,
-0.0202608444,
-0.02449104,
-0.146030575,
0.1155523881,
0.0659442022,
0.2069481462,
-0.1051696688,
-0.1531255841,
-0.052385807,
0.1600600779,
0.5171225667,
-0.4261570275,
0.263912797,
0.0571803115,
-0.0798494667,
-0.1211640388,
0.3827717304,
-0.0130477194,
-0.4193400443,
0.1440355182,
0.0797206014,
-0.1925650537,
0.1210365221,
-0.3839595318,
-0.1830467731,
0.0228526257,
-0.1718223393,
0.2358083874,
-0.2428785115,
0.0101263355,
-0.2272457033,
-0.0457868688,
0.1608249545,
-0.1947693378,
-0.1069455296,
-0.1489477009,
-0.478672415,
0.7286792994,
-0.1285426319,
0.0494992174,
0.4333668649,
-0.2160495222,
-0.3962888718,
0.4247333407,
-0.2746762633,
-0.260584414,
0.3615232706,
-0.4956049323,
0.463319093,
0.3245587647,
0.1980674565,
0.0893637463,
-0.0297740679,
0.1201325431,
-0.3002185822,
0.2200371474,
0.207102716,
-0.2394649088,
-0.1902951151,
-0.0840352699,
0.1256893277,
-0.0978199169,
0.0546353087,
-0.0002777483,
0.0439177193,
0.304869622,
0.1535117328,
0.0284014903,
-0.2191171497,
0.1361131966,
0.2374195606,
-0.192817077,
-0.0950853378,
-0.1431473792,
-0.0327364244,
-0.2658728361,
-0.0368485413,
-0.4485417902,
-0.2092806399,
0.0502896719,
0.2111270428,
0.0256069172,
-0.2873523831,
0.0026615111,
0.2300602347,
0.1467435807,
0.2893282473,
-0.2135195434,
0.4315738678,
-0.4924365282,
0.1644487977,
0.1382876039,
-0.1706263721,
0.1656694859,
-0.0245365743,
-0.0424522646,
0.0380518548,
-0.2710112929,
0.1686792076,
-0.0360042565,
0.2886779904,
-0.0269569866,
0.1526559293,
-0.2425819039,
0.2272117585,
0.0727076605,
0.0035590418,
-0.0096561722,
0.1620016098,
-0.0982811749,
0.1219338551,
0.0100626843,
-0.0682208166,
0.0612162948,
0.1239877641,
-0.0029773011,
-0.0025335727,
-0.2458426654,
0.3253137767,
-0.2929026783,
-0.2020264566,
-0.0993837714,
-0.2407434434,
0.1680092812,
0.239751786,
-0.2445982695,
0.1981149167,
0.5945558548,
-0.1142944619,
-0.0673989058,
0.3340903521,
0.11194098,
-0.0784528628,
0.1370969564,
0.0903343186,
0.1073062196,
0.1641958207,
0.1526740491,
0.0173446629,
0.1243101135,
-0.0069752368,
0.2460541427,
0.1179323941,
-0.3268172145,
0.0067925598,
-0.2291563302,
-0.1677503288,
-0.1305985749,
0.1232897937,
-0.4932426512,
-0.0216219984,
-0.3262926638,
0.0318341926,
-0.2363894582,
-0.3483006358,
-0.6054053307,
-0.0629227981,
-0.1207659692,
-0.3933293521,
0.2839479148,
0.0013737376,
-0.2738997638,
-0.1414477378,
0.1626860648,
0.3985837102,
-0.024409309,
0.181314528,
-0.5563156009,
0.3898975551,
-0.3940705061,
0.0558721609,
-0.1074177399,
0.1637452245,
0.3210857809,
-0.35895437,
-0.0686535463,
-0.298455894,
-0.0329045914,
0.1503030509,
-0.1243848279,
0.384601146,
0.1836295724,
0.1446369439,
0.1930282265,
-0.1698113829,
-0.114000231,
-0.2072125822,
-0.0516147166,
-0.2044170648,
0.2338192761,
0.1644958258,
-0.3444997668,
-0.3177578151,
-0.2811038196,
-0.2852053642,
0.1395975649,
-0.2266307473,
0.3257316351,
-0.3604023755,
-0.0258163381,
0.2454823405,
-0.3515591621,
0.5465461612,
0.1042626426,
-0.747580409,
-0.2174993604,
0.0302953422,
-0.0597591065,
-0.2551207244,
0.4536778629,
-0.2607752085,
-0.0767369121,
-0.7462229133,
0.0660567731,
-0.2379439771,
-0.1159114838,
0.3059663177,
-0.0538374372,
0.1463971287,
0.1931363642,
-0.061252363,
0.0539655276,
0.1244837269,
0.0279822312,
-0.4099283516,
0.3065108359,
0.2965652943,
0.189391017,
0.0822208077,
0.2465165555,
0.28100425,
0.0852203444,
-0.237820074,
-0.0867358893,
0.7142139077,
-0.3417423069,
-0.1789518297,
0.1240452901,
0.0556167997,
-0.3776141703,
0.1220379174,
-0.1001809388,
0.3989617229,
0.1636795551,
0.1444732994,
0.0491138883,
-0.3692816496,
0.0547100939,
0.0996537581,
-0.0200874079,
-0.0651740283,
0.050622385,
0.2321877927,
0.0743869618,
0.0726087242,
0.6089344621,
0.2598012686,
0.3592444956,
-0.3870895505,
-0.3552199602,
-0.5714656711,
-0.1087384,
0.1692545861,
0.043636255,
-0.4236885905,
-0.0780555978,
0.3002096713,
0.0457754992,
0.4229607582,
0.1064219624,
-0.0676106587,
-0.2087320536,
0.0002408623,
0.1899524331,
0.0841223225,
-0.2580263317,
0.2130457461,
0.4400644004,
-0.2385074198,
-0.3429697156,
-0.2365407944,
0.308786124,
-0.2244270742,
-0.0891736671,
-0.2934219539,
-0.369019419,
-0.0788290575,
-0.2005901784,
-0.0087905498,
0.1715461761,
0.1133401319,
-0.583822906,
-0.3357169032,
0.3812632561,
0.3573177755,
0.0346928425,
-0.2963632047,
0.0773243308,
-0.254717052,
-0.2744428217,
0.1503992975,
0.6171966791,
0.4491704404,
0.2272642553,
0.3225548565,
-0.0342023298,
0.0128655238,
-0.2113779038,
0.2160160393,
0.2802975774,
-0.263466388,
0.0105419522,
-0.0466081984,
0.2632806897,
-0.1785394847,
0.1241727918,
0.4459398985,
0.1868676841,
-0.2318394184,
-0.3095211089,
0.0230618771,
0.0784421414,
0.0268970616,
0.3604997694,
0.1483640969,
-0.1881167442,
-0.0462066904,
0.0034315065,
1.1015582085,
-0.1373367608,
0.260990411,
0.2877697647,
-0.3302657902,
0.3510972261,
0.1281823665,
0.0136729404,
-0.131152913,
-0.0354514569,
0.064399153,
-0.2890270352,
0.1884388775,
-0.0490273088,
-0.1270768791,
-0.0428867973,
0.021585539,
-0.0415109247,
0.0800618008,
0.102390416,
-0.216044873,
0.1060844511,
-0.2881344259,
0.0192072503,
-0.026541343,
-0.0139210494,
-0.1174460426,
0.0361349434,
0.1522875428,
-0.3208291233,
-0.1644946486,
-0.3527445495,
0.5632611513,
-0.1109792888,
0.0264697373,
0.1226060465,
-0.0527876168,
0.3851516545,
0.0218641739,
0.2955079377,
0.0929024145,
-0.4084981382,
-0.0887895674,
0.1000251621,
0.1308555007,
0.0152941663,
0.174143225,
-0.0800393894,
-0.3821747899,
0.185817197,
-0.0296149924,
-0.3801407516,
0.2591859698,
-0.2220319062,
0.1948300302,
-0.3954454064,
0.0477758944,
0.0720042214,
-0.1736709923,
0.0508769974,
0.097569041,
0.3021852076,
-0.2064762115,
0.2860215604,
-0.3368219137,
-0.6666277647,
-0.226028502,
0.1795530915,
0.174195677,
-0.0506714694,
0.2291257828,
-0.0015558217,
-0.4376497865,
-0.1027030125,
0.1720991582,
-0.0048596379,
-0.1171882674,
0.1323745251,
0.2386434376,
-0.0581312068,
-0.3001345694,
0.3246011138,
-0.0627130717,
0.0210992433,
-0.4857230484,
-0.0362967588,
-0.4227104485,
0.227353245,
-0.1513468176,
0.4059915841,
0.1191443056,
-0.0322225727,
0.0361276194,
-0.2973766029,
-0.257032752,
-0.3470446765,
-0.2968785763,
-0.0136986542,
0.1911919713,
0.0763877928,
0.3403457105,
0.1696931869,
0.0262364391,
0.037167836,
-0.2174251527,
-0.1059134528,
0.1565163881,
0.1749452651,
-0.1161087006,
0.3147472143,
-0.0786361322,
-0.0577073619,
0.1212154403,
0.178086549,
0.6493930221,
0.3984889686,
0.0585939437,
0.0507430919,
0.1323218495,
0.1496216357,
-0.053216733,
0.1916861236,
0.0050911894,
0.0918294266,
-0.1048525646,
-0.0315282233,
-0.3787101805,
-0.1021005511,
0.402752459,
0.2205820233,
0.311481446,
0.4311889112,
0.4334312379,
-0.055876296,
0.0868912861,
-0.0006714443,
0.2163829505,
0.1681904793,
-0.0863657445,
-0.3955234885,
-0.1428178102,
0.0818754211,
0.2249925882,
0.0101318592,
-0.344075501,
-0.2673564255,
-0.2052143216,
0.3394453824,
-0.0800556764,
-0.0660983473,
0.5081415176,
0.0618029982,
0.2550144494,
-0.1932758838,
-0.1969994754,
0.0877111852,
-0.3469739556,
0.2466040403,
0.3824838996,
0.2974499762,
-0.1563684642,
-0.102245003,
-0.0636557639,
0.1238488853,
0.1860052347,
-0.0362979062,
-0.1381464601,
0.080237098,
0.0349017084,
0.4141361713,
-0.1623231024,
-0.0629572496,
0.1144634411,
-0.248287499,
-0.0862406567,
0.1089331806,
0.0779775605,
-0.1425807029,
-0.2707242668,
0.1697159708,
-0.1490854472,
-0.0106326295,
-0.0939106122,
-0.0192940664,
0.030402964,
0.0467406549,
-0.158387363,
-0.0355548412,
0.07075385,
-0.2346913069,
-0.2739594281,
-0.2087141722,
0.0997998267,
-0.0873740986,
-0.0136820758,
-0.0403722301,
0.4138676822,
0.4520376325,
0.3165357411,
0.4676986337,
0.2450834513,
0.5179718733,
0.0220566858,
-0.1365852207,
0.0280263182,
-0.2476313263,
0.4332348704,
-0.0208044536,
-0.108908847,
0.3140865862,
0.0920557082,
-0.0360753797,
0.1127450988,
-0.0555472858,
0.2130410373,
-0.3242705464,
0.7055281997,
0.0282984711,
-0.2022134215,
-0.2135060728,
0.0174818411,
-0.5186846852,
-0.0205284785,
0.1742928177,
-0.1370123178,
0.0333148316,
-0.4020623267,
0.0729153529,
0.1446975768,
0.4893354475,
0.6453390718,
0.3660604656,
-0.1532875746,
-0.0984082669,
-0.1929562092,
0.2629055679,
0.1571943164,
0.0401858464,
-0.0300217085,
-0.1838282496,
-0.0041460576,
0.3262838721,
0.1542159915,
-0.2445581406,
-0.5066197515,
-0.0445621945,
0.0106719751,
0.0687693581,
0.3976646662,
0.2572368979,
-0.16429694,
-0.0032929513,
0.5116842985,
-0.2611890137,
-0.0154583361,
0.1191211045,
0.3817168474,
-0.1467203796,
-0.2076236606,
0.0127187753,
0.2066597492,
-0.0640814453,
0.0247611701,
-0.3828259706,
-0.0304994546,
0.0328761265,
-0.13187325,
-0.0021375078,
-0.2604813278,
0.4260803163,
-0.0194155518,
-0.0970738456,
-0.3136410713,
0.1424209625,
0.1574996561,
0.1751760989,
0.2302173674,
-0.1871323287,
-0.2503662407,
-0.0390241183,
0.2734517753,
0.2703129947,
0.350410372,
-0.0574521199,
-0.1396736503,
-0.1904395968,
0.359577179,
-0.1550253779,
-0.2110392153,
-0.1184630543,
0.2866292,
0.1683882177,
-0.1935352236,
-0.5391811728,
-0.0296886861,
0.2199974805,
-0.2367364466,
0.1419857889,
0.0874835849,
-0.398054868,
0.2825974524,
-0.019119082,
0.4385913908,
-0.2042134851,
0.340564549,
-0.1298887134,
-0.5378352404
]
|
https://github.com/huggingface/datasets/issues/2003 | Messages are being printed to the `stdout` | @lhoestq, sorry for the late reply
I completely understand why you decided to output a message that is always shown. The only problem is that the message is printed to the `stdout`. For example, if the user runs `python run_glue.py > log_file`, it will redirect `stdout` to the file named `log_file`, and the message will not be shown to the user.
Instead, we should print this message to `stderr`. Even in the case of `python run_glue.py > log_file` only `stdout` is being redirected and so the message is always shown. | In this code segment, we can see some messages are being printed to the `stdout`.
https://github.com/huggingface/datasets/blob/7e60bb509b595e8edc60a87f32b2bacfc065d607/src/datasets/builder.py#L545-L554
According to the comment, it is done intentionally, but I don't really understand why don't we log it with a higher level or print it directly to the `stderr`.
In my opinion, this kind of messages should never printed to the stdout. At least some configuration/flag should make it possible to provide in order to explicitly prevent the package to contaminate the stdout.
| 90 | Messages are being printed to the `stdout`
In this code segment, we can see some messages are being printed to the `stdout`.
https://github.com/huggingface/datasets/blob/7e60bb509b595e8edc60a87f32b2bacfc065d607/src/datasets/builder.py#L545-L554
According to the comment, it is done intentionally, but I don't really understand why don't we log it with a higher level or print it directly to the `stderr`.
In my opinion, this kind of messages should never printed to the stdout. At least some configuration/flag should make it possible to provide in order to explicitly prevent the package to contaminate the stdout.
@lhoestq, sorry for the late reply
I completely understand why you decided to output a message that is always shown. The only problem is that the message is printed to the `stdout`. For example, if the user runs `python run_glue.py > log_file`, it will redirect `stdout` to the file named `log_file`, and the message will not be shown to the user.
Instead, we should print this message to `stderr`. Even in the case of `python run_glue.py > log_file` only `stdout` is being redirected and so the message is always shown. | [
0.05412516,
-0.4283030629,
-0.017841002,
0.1863197088,
0.1776288301,
-0.1547812074,
0.3838961124,
0.1615998596,
0.0672425106,
0.2444253117,
0.1907832325,
0.3053063154,
-0.142559588,
0.284976095,
0.2610239387,
0.141788736,
-0.0724064931,
0.0228821728,
-0.4255485535,
0.1076408252,
-0.0089997258,
0.1110259667,
0.1996386349,
0.4757937789,
-0.6451058984,
-0.0969339386,
0.2199248523,
0.0682550073,
-0.1504666358,
-0.518029213,
-0.0038767331,
0.1959686279,
-0.0711478069,
0.2187255472,
-0.0001107846,
0.1114449054,
0.5583910942,
0.1802079976,
-0.5341905355,
-0.1494894326,
-0.0687195286,
-0.2411947995,
0.3232271075,
-0.138563782,
-0.1318151355,
-0.2632325292,
0.271494627,
-0.3419892788,
0.3885955215,
0.2979874909,
0.218565762,
0.2383881807,
0.0107336612,
0.2430755198,
0.1254063845,
0.2416488081,
0.0376769975,
-0.0428682715,
-0.0429108776,
0.3080662191,
-0.2036430687,
0.4286543131,
-0.0826229006,
-0.2804138958,
0.0262904726,
0.1417251676,
0.1627259403,
-0.3487530947,
0.0097639887,
0.0993573666,
0.2654751539,
-0.4483453333,
-0.1097154766,
-0.3162624836,
-0.0963750556,
-0.117158778,
0.1848395765,
0.1446038038,
-0.1712841839,
0.3809221387,
-0.1902453601,
-0.2524471879,
-0.2295150757,
-0.0734091401,
-0.1247814298,
0.0199616235,
-0.3208536804,
0.2482613027,
-0.221821636,
0.2160469294,
-0.185730055,
-0.2165313512,
-0.1245652065,
-0.0516974404,
-0.0614770055,
0.0057034274,
0.1151549965,
-0.0911647007,
-0.011603049,
-0.1248356476,
-0.2013801038,
0.0620360374,
0.2232738137,
0.1918917596,
0.488424778,
0.0879195407,
0.5125945807,
0.2814556062,
0.1908000559,
-0.042131979,
0.0905307904,
-0.2021926343,
0.308891505,
0.1708644629,
0.2888863385,
-0.2328936905,
0.2866508663,
-0.0900235847,
-0.0649688244,
0.1431060731,
-0.0611832403,
0.0710892677,
-0.0234852601,
0.2419445813,
0.0153703373,
-0.1141118109,
0.3781524003,
-0.0502082445,
0.0626935661,
-0.0070136241,
-0.1976067573,
0.0261097886,
-0.3317316771,
0.0355128944,
-0.1024648696,
-0.0499895625,
0.0674199387,
0.0976912528,
0.1535893679,
-0.1075142324,
-0.1262115389,
-0.0576670133,
0.4185281992,
0.4810257554,
-0.2644609511,
0.2954938412,
0.1943541467,
-0.2578786314,
-0.1323117912,
0.1952915639,
0.077141203,
-0.2959942222,
0.1112999842,
0.1830358505,
-0.3067575693,
0.323433578,
-0.21313788,
0.0265496597,
-0.0012508321,
-0.1195106804,
0.2357188761,
-0.1698852032,
-0.0174305867,
-0.2179443091,
0.1805112064,
0.3643778563,
-0.2229032665,
-0.1052752882,
-0.077198714,
-0.4211504161,
0.3274554014,
-0.2325009257,
0.1644018292,
0.3366905153,
-0.393691659,
-0.325060308,
0.4573613107,
-0.3337571323,
-0.1708880961,
0.2365000546,
-0.4048209786,
0.4652930796,
0.2076697797,
0.0043008015,
-0.005994909,
0.0376349613,
0.1742017269,
-0.1800449789,
0.3051739633,
0.1302235126,
-0.2291007936,
-0.0451015234,
-0.1515161842,
-0.0034163208,
-0.0958903432,
-0.0259336252,
-0.0169937219,
-0.1171618775,
0.4299753904,
0.2623740137,
0.07724794,
-0.2361661345,
0.2408174127,
0.2613837123,
-0.068564333,
-0.0481192358,
-0.0634817183,
0.0062269527,
-0.16583018,
0.0544400178,
-0.3115946054,
-0.221998632,
0.1507134885,
0.1317087263,
-0.1692024618,
-0.302621007,
0.1607027799,
0.2380981445,
0.1132998392,
0.302678138,
-0.119846195,
0.5206784606,
-0.3534439802,
0.0884948373,
-0.1319672763,
-0.0621948168,
0.2574378252,
-0.1281385124,
-0.0756491721,
0.2081560791,
-0.0934104025,
0.1671346426,
0.03816697,
0.4232922196,
-0.0043454189,
0.1183206216,
-0.3124952316,
0.050070934,
0.0097988443,
-0.0047036926,
0.0895716622,
0.1420447975,
-0.1465669125,
0.1471802443,
0.0384486951,
-0.0887250081,
0.1721268147,
0.150696829,
-0.0438550711,
0.0488442443,
-0.1227513328,
0.1988381594,
-0.4711334705,
-0.3036488891,
-0.2785584629,
-0.1713647842,
0.303311646,
0.1184072122,
-0.3035776317,
0.2379685342,
0.7682510614,
-0.030136751,
0.0205503982,
0.0657913014,
-0.2529128194,
0.0181570835,
0.1102728397,
0.2093631029,
0.1760624945,
0.2615463138,
0.2001334429,
0.0508064888,
0.0062752105,
0.0126905944,
0.2241540104,
0.06510932,
-0.2292537093,
0.0869501829,
-0.0851280838,
-0.2262379229,
-0.2331649214,
0.2796397507,
-0.5140918493,
-0.1945188642,
-0.3919094205,
0.0182173438,
-0.2353742272,
-0.403313458,
-0.5682979822,
-0.0971948281,
-0.0938996002,
-0.3950073123,
0.3544941545,
-0.0493450388,
-0.2513551712,
0.0566961542,
0.3190594614,
0.2767201364,
0.0284165014,
0.1718168855,
-0.4514870942,
0.2630798519,
-0.4042065144,
0.054278899,
-0.2848893106,
0.1247037649,
0.3621221185,
-0.2116228938,
-0.0718007162,
-0.2785490155,
-0.0587098375,
0.2219149321,
-0.2349594086,
0.5601468086,
0.304100275,
0.0496971011,
0.24474971,
-0.1081944481,
0.0988115817,
-0.3349266946,
-0.0422322378,
-0.2306945622,
0.1663374752,
0.1885209531,
-0.3057378232,
-0.249279961,
-0.3592654169,
-0.3399184942,
0.2599511743,
-0.2132179588,
0.2335206568,
-0.1511965841,
-0.1123752668,
0.1376271695,
-0.3935933411,
0.4972855747,
-0.088185139,
-0.6302130818,
-0.1551143527,
-0.1244043708,
-0.090823099,
-0.1654583663,
0.1136174351,
-0.2327756733,
-0.2250758559,
-0.560444057,
-0.293710798,
-0.2211568803,
-0.1356342733,
0.26473701,
0.3401816487,
0.1737588793,
0.1155197769,
-0.1217629015,
-0.0775535926,
-0.0946767703,
0.0891972929,
-0.3017316163,
0.2018404156,
0.2314454466,
0.1525158286,
0.0308499392,
0.1316173077,
0.3769788742,
0.123182714,
-0.2599918246,
-0.1977273226,
0.9344840646,
-0.3076796532,
-0.1384756267,
-0.0149094556,
0.1217962578,
-0.3135746717,
0.1371773183,
-0.0295711607,
0.4288764894,
0.1746638417,
0.0135242417,
-0.1403274238,
-0.4702349007,
-0.0877083763,
0.0079169357,
-0.1061838865,
-0.03829493,
0.0241899192,
0.2270082533,
0.10133183,
-0.0078089,
0.4861996472,
0.3321849108,
0.3255207241,
-0.2572868168,
-0.3790224493,
-0.4488612711,
-0.108459726,
-0.1151636094,
-0.0170604009,
-0.284850955,
-0.3079067171,
0.301550597,
0.1109930649,
0.4875507355,
-0.0799731016,
0.0736555904,
-0.0477081165,
0.039941255,
-0.0594903193,
-0.0044632475,
-0.2306836694,
0.2239956558,
0.3898358047,
-0.0665588528,
-0.2347534746,
-0.2896490097,
0.3323348761,
-0.1647359431,
-0.2118755281,
-0.3469476998,
-0.4939077497,
0.0554592125,
-0.070800595,
0.1633123457,
0.198954463,
-0.0298538655,
-0.4865507782,
-0.463731885,
0.2801905274,
0.11292959,
0.0338786282,
-0.0750611722,
0.2129461914,
-0.2921411097,
-0.215669021,
0.3172506094,
0.5881593823,
0.0688565373,
0.20732072,
0.0794396475,
0.088109456,
0.0209727194,
-0.2785409093,
0.3618935049,
0.2727496326,
-0.2944972217,
0.0256185103,
-0.2036677152,
0.2632232308,
-0.229089886,
0.0429352261,
0.3444984853,
0.2117917091,
-0.1790156066,
-0.3018656373,
0.0961392894,
-0.0411254466,
-0.1296868622,
0.3108429611,
0.2002138495,
-0.3194751143,
0.0456755683,
-0.1375167072,
0.9276249409,
-0.0491976663,
0.2219361365,
0.2238164693,
-0.2233252078,
0.3959762752,
0.0447029248,
0.1350616068,
-0.2658404112,
-0.1578317434,
0.1012428254,
-0.2373302728,
-0.0095097218,
0.0341934785,
-0.1483631134,
0.0392885432,
0.0145298596,
-0.0474168807,
-0.0873331279,
0.1859937161,
-0.1557832509,
0.0478832908,
-0.4868291616,
0.0810600221,
0.1670163274,
-0.0533472635,
-0.0447651483,
0.0376884975,
0.4169226885,
-0.3255186975,
-0.0336936191,
-0.2197087705,
0.2313966453,
-0.1718176454,
0.1313593388,
0.0232820809,
-0.1901387721,
0.220040381,
-0.0098537384,
0.5312998295,
0.227298528,
-0.3040036857,
0.2454186082,
0.1460797787,
-0.0669892654,
0.1092392802,
0.3509555459,
0.0825151727,
-0.4282552898,
0.2340438515,
-0.046178896,
-0.2830711901,
0.075105533,
-0.0555657558,
0.1881590337,
-0.3063421845,
0.1682014465,
0.1003827825,
-0.0473899543,
0.0465491079,
0.1458730847,
0.3736650348,
-0.3556674719,
0.3597013354,
-0.2477941811,
-0.642005682,
-0.0725755915,
-0.002232156,
0.1410233527,
0.1321802735,
0.1211477816,
0.0531026907,
-0.3038239181,
-0.1832688004,
0.0849186182,
0.243080914,
0.0785036609,
0.1308674961,
0.3694795966,
-0.0232785363,
-0.0923412293,
0.1055510193,
-0.1175148413,
-0.043843288,
-0.5810170174,
-0.1720309556,
-0.291728586,
0.2343804836,
-0.1726211011,
0.3928423524,
0.33715716,
-0.0697138682,
0.1000315025,
-0.0485727601,
-0.3665170372,
-0.3736621439,
-0.1796022803,
0.1179321036,
0.2822410762,
0.0690289736,
0.3685358763,
0.0198376421,
0.1314407736,
-0.0938631967,
-0.2340659797,
-0.2157687694,
0.1162944213,
0.1149277613,
-0.1505063325,
0.3382874727,
-0.1192692891,
-0.1658317,
0.2831535637,
0.0107910996,
0.8302780986,
0.380509913,
-0.0503564626,
0.079723455,
0.0365441069,
0.0940267965,
-0.0143631864,
0.1291986853,
0.0394771099,
-0.1125791967,
-0.0027946192,
-0.0728811026,
-0.4296863079,
-0.0618371107,
0.4453522861,
0.2021914423,
0.1739316583,
0.5326746702,
0.1915153712,
-0.0546603687,
-0.1256483495,
-0.237899527,
0.4046914279,
0.0986345261,
-0.0769896358,
-0.4025781751,
-0.1153150797,
0.1952557266,
0.0609416813,
0.0851305127,
-0.1067547947,
-0.3498921394,
0.0727883577,
0.3925573528,
0.018866986,
-0.0715334266,
0.4490740895,
0.140565455,
0.1830878556,
-0.3522501886,
-0.1274861842,
0.0602806509,
-0.3531312048,
0.1583716124,
0.4669976234,
0.1810621023,
-0.1216160655,
0.0250403099,
0.1749841869,
0.1975759566,
0.232255578,
-0.1198538095,
-0.0334319286,
0.1030742079,
0.0993199944,
0.2516612113,
-0.2458587587,
-0.0670951381,
-0.0377258956,
0.0330496654,
-0.2389989197,
0.1359067261,
0.0128853293,
-0.1712244749,
-0.186976254,
0.1009697691,
0.006852176,
0.0185961053,
-0.1414889246,
-0.1694045067,
0.2064243257,
-0.07986781,
-0.0593570955,
-0.0337696522,
0.108221963,
-0.1675758213,
-0.2668061852,
-0.1747729778,
0.0726370662,
-0.0165564269,
0.14556548,
-0.1115005165,
0.4508195519,
0.352121979,
0.2865578532,
0.3099736571,
0.1852802932,
0.3356394172,
0.0134089785,
-0.1203371584,
-0.0764830261,
-0.3353626728,
0.3160896599,
-0.012912713,
-0.2447959632,
0.1927162111,
0.2330978364,
-0.1097832695,
0.0718367025,
-0.0239259861,
0.0817840621,
-0.2276335061,
0.6042563319,
0.1579877436,
-0.2104890496,
-0.252617389,
-0.0216336362,
-0.6160032153,
-0.1130649596,
0.4817125499,
-0.0899932459,
-0.0288040359,
-0.5377420187,
0.1322620213,
0.1168790087,
0.2316970974,
0.4534965456,
0.3030887246,
0.0223233663,
0.0053227097,
-0.2670534849,
0.3397336304,
0.097447522,
-0.1241302192,
-0.1588825881,
-0.115259245,
0.020044798,
0.1332343519,
0.2141839266,
-0.0767310932,
-0.4619906247,
-0.2051265985,
-0.0448316559,
0.036786709,
0.357684195,
0.1559444666,
-0.0222838819,
-0.0010506192,
0.2269449085,
-0.2366587371,
0.0921263769,
0.304031074,
0.3641933203,
-0.0291608777,
-0.1873097569,
0.2222923189,
0.2810075581,
0.060316626,
-0.0935687274,
-0.1979131252,
-0.1583642364,
0.0454438329,
-0.3171055019,
0.0281598549,
-0.1240471005,
0.4336729646,
-0.0226713438,
-0.2668975294,
-0.2442458421,
0.0407405272,
0.2229071558,
0.0435874909,
0.3966625035,
-0.0439385772,
-0.1420144737,
-0.00847496,
0.1539935768,
0.3463276923,
0.258829236,
-0.0758059993,
-0.0459531322,
-0.2183857709,
0.2991535962,
-0.3736028969,
-0.3177956045,
-0.1893787235,
0.3814631999,
0.095850192,
-0.1871169955,
-0.5491604805,
-0.1038086936,
0.2456925511,
-0.1885931492,
0.1300996393,
0.1423919648,
-0.3548879027,
0.2615863979,
-0.0409366526,
0.2532685101,
-0.0565302372,
0.2077295333,
-0.071963191,
-0.5686920285
]
|
https://github.com/huggingface/datasets/issues/2000 | Windows Permission Error (most recent version of datasets) | Hi @itsLuisa !
Could you give us more information about the error you're getting, please?
A copy-paste of the Traceback would be nice to get a better understanding of what is wrong :) | Hi everyone,
Can anyone help me with why the dataset loading script below raises a Windows Permission Error? I stuck quite closely to https://github.com/huggingface/datasets/blob/master/datasets/conll2003/conll2003.py , only I want to load the data from three local three-column tsv-files (id\ttokens\tpos_tags\n). I am using the most recent version of datasets. Thank you in advance!
Luisa
My script:
```
import datasets
import csv
logger = datasets.logging.get_logger(__name__)
class SampleConfig(datasets.BuilderConfig):
def __init__(self, **kwargs):
super(SampleConfig, self).__init__(**kwargs)
class Sample(datasets.GeneratorBasedBuilder):
BUILDER_CONFIGS = [
SampleConfig(name="conll2003", version=datasets.Version("1.0.0"), description="Conll2003 dataset"),
]
def _info(self):
return datasets.DatasetInfo(
description="Dataset with words and their POS-Tags",
features=datasets.Features(
{
"id": datasets.Value("string"),
"tokens": datasets.Sequence(datasets.Value("string")),
"pos_tags": datasets.Sequence(
datasets.features.ClassLabel(
names=[
"''",
",",
"-LRB-",
"-RRB-",
".",
":",
"CC",
"CD",
"DT",
"EX",
"FW",
"HYPH",
"IN",
"JJ",
"JJR",
"JJS",
"MD",
"NN",
"NNP",
"NNPS",
"NNS",
"PDT",
"POS",
"PRP",
"PRP$",
"RB",
"RBR",
"RBS",
"RP",
"TO",
"UH",
"VB",
"VBD",
"VBG",
"VBN",
"VBP",
"VBZ",
"WDT",
"WP",
"WRB",
"``"
]
)
),
}
),
supervised_keys=None,
homepage="https://catalog.ldc.upenn.edu/LDC2011T03",
citation="Weischedel, Ralph, et al. OntoNotes Release 4.0 LDC2011T03. Web Download. Philadelphia: Linguistic Data Consortium, 2011.",
)
def _split_generators(self, dl_manager):
loaded_files = dl_manager.download_and_extract(self.config.data_files)
return [
datasets.SplitGenerator(name=datasets.Split.TRAIN, gen_kwargs={"filepath": loaded_files["train"]}),
datasets.SplitGenerator(name=datasets.Split.TEST, gen_kwargs={"filepath": loaded_files["test"]}),
datasets.SplitGenerator(name=datasets.Split.VALIDATION, gen_kwargs={"filepath": loaded_files["val"]})
]
def _generate_examples(self, filepath):
logger.info("generating examples from = %s", filepath)
with open(filepath, encoding="cp1252") as f:
data = csv.reader(f, delimiter="\t")
ids = list()
tokens = list()
pos_tags = list()
for id_, line in enumerate(data):
#print(line)
if len(line) == 1:
if tokens:
yield id_, {"id": ids, "tokens": tokens, "pos_tags": pos_tags}
ids = list()
tokens = list()
pos_tags = list()
else:
ids.append(line[0])
tokens.append(line[1])
pos_tags.append(line[2])
# last example
yield id_, {"id": ids, "tokens": tokens, "pos_tags": pos_tags}
def main():
dataset = datasets.load_dataset(
"data_loading.py", data_files={
"train": "train.tsv",
"test": "test.tsv",
"val": "val.tsv"
}
)
#print(dataset)
if __name__=="__main__":
main()
```
| 33 | Windows Permission Error (most recent version of datasets)
Hi everyone,
Can anyone help me with why the dataset loading script below raises a Windows Permission Error? I stuck quite closely to https://github.com/huggingface/datasets/blob/master/datasets/conll2003/conll2003.py , only I want to load the data from three local three-column tsv-files (id\ttokens\tpos_tags\n). I am using the most recent version of datasets. Thank you in advance!
Luisa
My script:
```
import datasets
import csv
logger = datasets.logging.get_logger(__name__)
class SampleConfig(datasets.BuilderConfig):
def __init__(self, **kwargs):
super(SampleConfig, self).__init__(**kwargs)
class Sample(datasets.GeneratorBasedBuilder):
BUILDER_CONFIGS = [
SampleConfig(name="conll2003", version=datasets.Version("1.0.0"), description="Conll2003 dataset"),
]
def _info(self):
return datasets.DatasetInfo(
description="Dataset with words and their POS-Tags",
features=datasets.Features(
{
"id": datasets.Value("string"),
"tokens": datasets.Sequence(datasets.Value("string")),
"pos_tags": datasets.Sequence(
datasets.features.ClassLabel(
names=[
"''",
",",
"-LRB-",
"-RRB-",
".",
":",
"CC",
"CD",
"DT",
"EX",
"FW",
"HYPH",
"IN",
"JJ",
"JJR",
"JJS",
"MD",
"NN",
"NNP",
"NNPS",
"NNS",
"PDT",
"POS",
"PRP",
"PRP$",
"RB",
"RBR",
"RBS",
"RP",
"TO",
"UH",
"VB",
"VBD",
"VBG",
"VBN",
"VBP",
"VBZ",
"WDT",
"WP",
"WRB",
"``"
]
)
),
}
),
supervised_keys=None,
homepage="https://catalog.ldc.upenn.edu/LDC2011T03",
citation="Weischedel, Ralph, et al. OntoNotes Release 4.0 LDC2011T03. Web Download. Philadelphia: Linguistic Data Consortium, 2011.",
)
def _split_generators(self, dl_manager):
loaded_files = dl_manager.download_and_extract(self.config.data_files)
return [
datasets.SplitGenerator(name=datasets.Split.TRAIN, gen_kwargs={"filepath": loaded_files["train"]}),
datasets.SplitGenerator(name=datasets.Split.TEST, gen_kwargs={"filepath": loaded_files["test"]}),
datasets.SplitGenerator(name=datasets.Split.VALIDATION, gen_kwargs={"filepath": loaded_files["val"]})
]
def _generate_examples(self, filepath):
logger.info("generating examples from = %s", filepath)
with open(filepath, encoding="cp1252") as f:
data = csv.reader(f, delimiter="\t")
ids = list()
tokens = list()
pos_tags = list()
for id_, line in enumerate(data):
#print(line)
if len(line) == 1:
if tokens:
yield id_, {"id": ids, "tokens": tokens, "pos_tags": pos_tags}
ids = list()
tokens = list()
pos_tags = list()
else:
ids.append(line[0])
tokens.append(line[1])
pos_tags.append(line[2])
# last example
yield id_, {"id": ids, "tokens": tokens, "pos_tags": pos_tags}
def main():
dataset = datasets.load_dataset(
"data_loading.py", data_files={
"train": "train.tsv",
"test": "test.tsv",
"val": "val.tsv"
}
)
#print(dataset)
if __name__=="__main__":
main()
```
Hi @itsLuisa !
Could you give us more information about the error you're getting, please?
A copy-paste of the Traceback would be nice to get a better understanding of what is wrong :) | [
-0.1825570911,
0.1823853403,
-0.0400311947,
0.2590482235,
0.0813781247,
0.1336375624,
0.4580341578,
0.0122546703,
0.1864631921,
0.0741284192,
-0.0634553432,
-0.0112266093,
-0.1087960601,
0.1572923511,
-0.0323619768,
-0.0282509699,
0.1453508139,
0.0480683856,
0.0319975466,
0.139645949,
-0.4052310884,
-0.007121121,
-0.2379361093,
0.1073664501,
-0.2231619209,
0.2783439457,
-0.0157265253,
0.3908287287,
-0.0338029079,
-0.2350180447,
0.2101651728,
0.3624859452,
0.6620944142,
0.2453529537,
-0.0001126285,
-0.0484501161,
0.0420434475,
-0.0201319195,
0.2068192661,
0.2423871309,
0.1341382414,
-0.04518703,
0.0041251788,
-0.1066692024,
-0.1698370129,
0.2000027448,
-0.0654299706,
-0.3538348079,
0.1139975935,
0.4263343811,
0.1871958673,
0.247462675,
-0.1806876957,
-0.0106539074,
0.5910393596,
0.0475985892,
-0.1261596382,
0.1927935481,
0.2900242507,
-0.4428054094,
0.2141413689,
0.1609823704,
-0.2268586606,
0.0855402499,
0.3818269372,
-0.1632637531,
-0.1299572885,
-0.283038944,
0.1584062576,
0.0000530392,
0.7270632982,
-0.1692434996,
-0.3009923398,
-0.0341044217,
0.1393089592,
-0.2671693265,
0.2275046855,
0.3365988135,
-0.050527174,
0.0753582194,
-0.2347000539,
-0.1671509296,
-0.443400681,
0.1358956397,
0.1775049269,
-0.2292980105,
-0.0849329084,
0.2297088653,
0.0906637385,
-0.1301722825,
0.3544328213,
0.1294435561,
0.0419616289,
0.2517597675,
-0.4671479762,
0.3360511959,
0.2092745006,
0.2980215251,
-0.0320270434,
0.2779500484,
-0.1361711621,
-0.2106274962,
0.2018986344,
0.1250197887,
0.1772743315,
-0.0542738587,
0.1519603282,
0.2411281615,
0.1375095099,
-0.02251116,
0.0402428508,
-0.1174408719,
-0.4900485575,
-0.6061115265,
0.1152088568,
0.2454816103,
0.3073365986,
-0.089267306,
-0.3613362312,
0.1164761037,
0.1181356385,
0.0680991709,
0.2068188041,
0.4097330272,
0.2663402855,
0.011945026,
0.3739323914,
0.2714996338,
-0.0628644973,
-0.0258000549,
-0.0862124264,
0.004246146,
-0.2458985001,
-0.0498456918,
0.4257870018,
-0.344258368,
-0.1134994254,
0.1173631102,
0.0353441276,
-0.1213496029,
0.0494541749,
-0.018771844,
0.1164599434,
0.438858062,
0.258418113,
0.0349972434,
0.1450433284,
-0.1851192862,
-0.0282775648,
0.2364420742,
-0.132414028,
-0.340477109,
-0.1883571893,
0.1011297554,
0.0170741994,
0.0499141589,
-0.2183059752,
-0.1330368519,
0.1228498742,
-0.018460894,
0.1442884654,
-0.1007024795,
-0.2660650909,
-0.3397949338,
-0.1015141979,
0.8314391375,
-0.5272638202,
0.1402403116,
-0.1147452071,
-0.304766953,
0.1072497815,
0.331196934,
-0.0764998272,
0.1113563403,
-0.2144830525,
0.1516457945,
-0.1357124448,
-0.368085146,
-0.1788970232,
0.3780058324,
-0.0177540891,
-0.1036801413,
0.2437340766,
-0.0390077457,
0.0444856919,
-0.2033563554,
-0.0898015723,
0.1341284364,
0.1384612024,
0.2605626285,
0.2249746919,
0.0075680506,
0.232209444,
0.312423408,
0.0553451143,
-0.0627512485,
0.2918674648,
-0.2186995149,
0.1895523965,
-0.3010882437,
0.2245456576,
0.4166682065,
0.1158196256,
0.3091973364,
0.0332214348,
-0.2322231084,
-0.4455741644,
0.2652017176,
0.3054820597,
-0.1239581332,
0.0469029248,
-0.2565839291,
-0.1502389908,
0.2109270543,
-0.3883989155,
0.1167707741,
0.0899114236,
0.2979707718,
-0.177402094,
-0.3208981156,
0.0345413946,
0.0563448817,
-0.2444297522,
-0.1111344323,
-0.1534329355,
0.03845869,
-0.3066702783,
0.0180421192,
0.2049439102,
-0.0148222595,
0.415769726,
-0.0743798465,
0.0026423864,
0.3591324985,
0.2803247273,
-0.0367781892,
0.0367961526,
0.0983091071,
-0.2261276245,
0.0771445036,
0.1272579283,
-0.0095818406,
0.0916863456,
-0.102523081,
-0.0255537909,
0.1265099198,
-0.2259501666,
0.0041123168,
-0.2879078686,
0.1314649731,
-0.0096178232,
0.0248836391,
-0.0246058516,
-0.2199263126,
0.3593811095,
0.5096368194,
-0.3285351992,
-0.0453587845,
-0.1026295051,
-0.0515074171,
0.2793928385,
-0.1505600214,
-0.1328615099,
0.0687072054,
0.0991908312,
0.2852438986,
0.1979035139,
0.2263827026,
0.4385740757,
0.0644595921,
-0.1653025001,
0.0529507995,
0.2032762915,
-0.0528066158,
0.1483860165,
-0.3353100419,
0.0793049783,
0.2603352368,
-0.299210012,
0.1275259107,
-0.3775517642,
-0.1403334588,
0.1440864801,
0.3369530141,
-0.5138479471,
-0.1326297224,
-0.0149591882,
-0.1334607005,
0.1105090231,
-0.3155809045,
-0.2831481993,
0.0130273448,
-0.2013535202,
0.162641421,
-0.2732631266,
-0.1256406903,
-0.4015874565,
0.0573597215,
0.2376945019,
-0.0031728656,
-0.0217247047,
0.0865614191,
-0.0238456167,
0.0146017903,
0.4932957292,
-0.28297472,
0.4370730221,
-0.1266709268,
0.3814795315,
-0.2898306549,
0.1060907543,
-0.2475937605,
-0.3344739377,
0.3203749657,
0.2462344468,
0.0983887985,
-0.0262449812,
-0.2058809102,
0.1195864901,
-0.1224582121,
-0.0811636224,
0.1755106896,
0.3042165041,
-0.5918186903,
-0.2506393492,
-0.1534151435,
-0.2042927295,
-0.3533948064,
0.2209897935,
0.1355350614,
0.3110540211,
0.2072858363,
-0.1218602806,
0.0530252792,
0.043456085,
0.3111527562,
-0.3197954595,
-0.0964616165,
0.0137316855,
-0.1502658129,
-0.5285491347,
0.2205064446,
0.3835148811,
-0.1217220724,
0.1091841757,
-0.1158390269,
0.125109762,
-0.2140982598,
0.2546712458,
-0.228287816,
0.1662581116,
0.1928659528,
0.0734915808,
0.0805567577,
-0.1694662571,
-0.0990941674,
-0.3450900316,
-0.2438754141,
-0.2646103203,
0.1542904377,
0.3330394924,
-0.131513983,
0.2149935961,
0.3426208198,
0.2264257967,
0.4461936951,
-0.1276844591,
0.4316770136,
0.2818518877,
-0.6437911391,
0.1521578133,
-0.18613033,
-0.05307265,
0.3601595163,
-0.0777109787,
0.0294725727,
-0.1574552804,
0.058306057,
-0.3378960192,
-0.2107928544,
-0.0072615365,
-0.2567558885,
0.2782018185,
-0.1479891986,
0.0072967792,
-0.2808843851,
-0.0628954396,
0.005472145,
0.5689313412,
0.1167745665,
-0.0616015047,
-0.3760633171,
0.0078707105,
-0.4856027961,
0.2696469426,
0.0624525286,
0.6091941595,
-0.1555263102,
0.0315031335,
0.1437780112,
0.1085257679,
0.9010461569,
-0.4391022623,
0.2763885856,
0.2481008172,
0.1166943014,
-0.2631775141,
-0.2115413994,
-0.2682220042,
0.0674349964,
-0.0131370639,
0.3030071557,
0.0118964575,
-0.3937036097,
0.0339969285,
-0.153051123,
-0.0794088542,
-0.4198120534,
-0.2535521388,
-0.1815235913,
-0.3771492243,
-0.104181312,
0.23979415,
0.1634427756,
-0.2563314438,
0.2497168928,
-0.0224106852,
0.2882218957,
0.1148119718,
-0.0406745709,
0.1016073227,
0.3072129786,
0.0786269903,
-0.0809461549,
0.1236321703,
0.2123339176,
0.5588173866,
-0.0261244364,
-0.3647989035,
-0.1107672825,
-0.0270075183,
0.4848946333,
0.0845979899,
-0.1533036828,
0.1014052853,
-0.2211104035,
-0.0586444661,
-0.3480155766,
0.0739531368,
0.2731053531,
-0.0515426248,
0.0595361367,
-0.2157611251,
0.3164066076,
0.1723265499,
-0.1943371892,
-0.0005693612,
0.1422990113,
-0.2947746515,
-0.0935067385,
0.0510760061,
0.5023057461,
-0.2940065563,
0.2797781825,
0.1955999881,
-0.1659104526,
-0.0711433366,
0.2135722637,
-0.0846961737,
-0.4017241597,
-0.3005034924,
-0.0134298494,
-0.1052670032,
-0.0054747174,
0.1585410684,
0.0849553198,
0.0400236696,
-0.0924143121,
0.3835963905,
0.0317606591,
0.0596931167,
-0.2020756453,
-0.2441046536,
0.1019106731,
0.1519687027,
-0.1361003816,
-0.0235723015,
-0.1311586946,
0.0135435546,
0.1534245759,
0.0239187609,
-0.3978717327,
0.2855560184,
-0.327996254,
0.010729772,
-0.1360234916,
0.0947397053,
0.5240967274,
0.4402703643,
-0.0064930753,
0.145454824,
-0.253116399,
-0.2666569054,
0.1540938616,
-0.2917835712,
-0.2915798128,
-0.0236649364,
0.2547367513,
0.0212812591,
-0.3019712567,
-0.1922041774,
-0.0331775397,
-0.243269071,
-0.0630601123,
0.4675464034,
0.1187869161,
-0.5070376396,
-0.1682939529,
-0.2320503443,
-0.0584002212,
0.0715505257,
0.1030867025,
0.1528619826,
-0.108543478,
0.061982438,
-0.2745188773,
-0.2699644566,
0.0699822605,
0.4720024765,
-0.275008291,
0.1021839082,
0.544580102,
0.4029548466,
-0.160260722,
-0.2423873544,
0.0846468583,
0.0800293684,
-0.2135887444,
0.0007552103,
0.3120194972,
0.0452675112,
0.4206766784,
0.0679823831,
0.2228616774,
-0.4020855725,
0.1897779852,
-0.4625520706,
-0.1102989763,
-0.0878792927,
-0.1858240962,
0.312094599,
0.0934093669,
-0.144258067,
0.0303128082,
-0.2334229499,
-0.2932747602,
0.1127860695,
-0.0025985059,
0.1187577322,
0.4522460699,
-0.2297088653,
-0.085651271,
-0.0219585076,
0.0636569858,
-0.1481773853,
-0.1781421304,
-0.2058388144,
0.0039552306,
0.1113950461,
0.1878647059,
-0.3387505114,
0.0858406574,
-0.5403543115,
-0.2182606459,
-0.1620230228,
0.2066287547,
0.2949579656,
-0.1138813123,
0.4738370776,
0.0064511574,
-0.0148760919,
0.0212340187,
0.1910280138,
-0.1915097386,
0.085947223,
-0.3789151311,
0.4132499099,
0.0258934665,
-0.085448429,
-0.3049194813,
0.2492911518,
0.2421406657,
0.1775054485,
0.1364677548,
-0.2938656211,
0.0452843755,
0.1257750988,
0.5298550725,
0.0940535814,
-0.3113822341,
0.030527601,
0.1404467821,
0.2037546486,
-0.3257119358,
-0.1571353972,
-0.2650842965,
-0.1184610054,
-0.0624470823,
0.1154166311,
0.011267364,
-0.3411979377,
-0.0892772675,
-0.1154887453,
0.2970601022,
0.2271779776,
-0.0939653441,
0.5441295505,
0.0009057375,
-0.0297026373,
0.3708810508,
0.5498603582,
0.0500367656,
0.4243816733,
0.1949298531,
-0.1030109748,
-0.1730054319,
0.0265197121,
-0.0050948379,
-0.7224724293,
0.1903757155,
-0.0895251706,
-0.0153278196,
0.0070984089,
-0.1846191734,
0.4962286949,
-0.1142301932,
-0.0175314061,
-0.3881717026,
0.1512206495,
-0.2028815299,
-0.1655405015,
-0.423861444,
0.0989476144,
-0.0877482966,
-0.0205381364,
-0.1252162457,
-0.246002242,
0.0037853292,
0.2284580171,
-0.032579381,
-0.2135649174,
0.3492715955,
0.2529511452,
-0.1378227323,
-0.3874363899,
-0.0780466571,
-0.0577865727,
0.120366253,
0.1329324841,
0.0222340617,
0.6245728731,
0.3318483531,
-0.0558945797,
-0.0126736993,
0.0129632708,
-0.0314593762,
-0.0356336199,
0.3866262138,
-0.2138805538,
0.3700493574,
0.3404142559,
0.0974965319,
-0.0588645488,
-0.0613858737,
0.039735008,
-0.1048274115,
-0.0849886015,
-0.0384140871,
-0.3763243258,
-0.2585603297,
-0.1624861807,
0.1023469418,
-0.0869154185,
-0.0223290734,
0.6900109053,
0.1503064334,
0.3419894278,
-0.3378277719,
0.0661960468,
0.0295752492,
0.4036604464,
0.4701581597,
0.0760131702,
-0.4037769139,
-0.0799949691,
-0.4968743622,
0.0621398464,
0.121179074,
-0.1317166239,
0.179227978,
-0.1361561269,
-0.0133228628,
-0.1985216886,
-0.0573431291,
0.0297258981,
0.0242386498,
0.3217082024,
-0.0086835288,
-0.1077248156,
0.265558064,
-0.0672237948,
0.142630592,
-0.5638712049,
0.0671391413,
-0.2215020061,
0.0575662628,
-0.4719405472,
0.0898200274,
0.123853825,
0.1396860182,
0.2431275547,
0.0155662708,
0.3771501482,
-0.0043459646,
0.1913797259,
-0.5739654899,
0.0678086728,
-0.1726516038,
0.143406868,
-0.1353252232,
-0.0251371469,
-0.2696663439,
0.0728009269,
-0.0983975381,
0.1188254878,
-0.0468874499,
0.1527617872,
-0.0512723923,
0.1282428652,
-0.1833433509,
-0.0416471846,
0.221635744,
-0.1437096447,
-0.1895446926,
0.3802647591,
-0.32337749,
-0.3723748326,
0.4683174789,
-0.2650414705,
-0.251288563,
0.0131053897,
0.3566475213,
-0.1201090291,
-0.1665671319,
-0.1439182609,
0.3625414073,
0.1724918336,
-0.0048149573,
-0.3380491436,
0.4713962674,
-0.203684479,
-0.1848140061,
0.0364144295,
0.423489362,
-0.0084359813,
-0.2079801559,
0.0341636352,
-0.1151267961
]
|
https://github.com/huggingface/datasets/issues/2000 | Windows Permission Error (most recent version of datasets) | Hello @SBrandeis , this is it:
```
Traceback (most recent call last):
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\site-packages\datasets\builder.py", line 537, in incomplete_dir
yield tmp_dir
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\site-packages\datasets\builder.py", line 578, in download_and_prepare
self._download_and_prepare(
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\site-packages\datasets\builder.py", line 656, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\site-packages\datasets\builder.py", line 982, in _prepare_split
num_examples, num_bytes = writer.finalize()
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\site-packages\datasets\arrow_writer.py", line 297, in finalize
self.write_on_file()
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\site-packages\datasets\arrow_writer.py", line 230, in write_on_file
pa_array = pa.array(typed_sequence)
File "pyarrow\array.pxi", line 222, in pyarrow.lib.array
File "pyarrow\array.pxi", line 110, in pyarrow.lib._handle_arrow_array_protocol
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\site-packages\datasets\arrow_writer.py", line 97, in __arrow_array__
out = pa.array(self.data, type=type)
File "pyarrow\array.pxi", line 305, in pyarrow.lib.array
File "pyarrow\array.pxi", line 39, in pyarrow.lib._sequence_to_array
File "pyarrow\error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow\error.pxi", line 107, in pyarrow.lib.check_status
pyarrow.lib.ArrowTypeError: Expected bytes, got a 'list' object
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "C:/Users/Luisa/Documents/Uni/WS 2020,21/Neural Networks/Final_Project/NN_Project/data_loading.py", line 122, in <module>
main()
File "C:/Users/Luisa/Documents/Uni/WS 2020,21/Neural Networks/Final_Project/NN_Project/data_loading.py", line 111, in main
dataset = datasets.load_dataset(
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\site-packages\datasets\load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\site-packages\datasets\builder.py", line 586, in download_and_prepare
self._save_info()
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\contextlib.py", line 131, in __exit__
self.gen.throw(type, value, traceback)
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\site-packages\datasets\builder.py", line 543, in incomplete_dir
shutil.rmtree(tmp_dir)
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\shutil.py", line 740, in rmtree
return _rmtree_unsafe(path, onerror)
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\shutil.py", line 618, in _rmtree_unsafe
onerror(os.unlink, fullname, sys.exc_info())
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\shutil.py", line 616, in _rmtree_unsafe
os.unlink(fullname)
PermissionError: [WinError 32] Der Prozess kann nicht auf die Datei zugreifen, da sie von einem anderen Prozess verwendet wird: 'C:\\Users\\Luisa\\.cache\\huggingface\\datasets\\sample\\default-20ee7d51a6a9454f\\0.0.0\\5fc4c3a355ea77ab446bd31fca5082437600b8364d29b2b95264048bd1f398b1.incomplete\\sample-train.arrow'
Process finished with exit code 1
``` | Hi everyone,
Can anyone help me with why the dataset loading script below raises a Windows Permission Error? I stuck quite closely to https://github.com/huggingface/datasets/blob/master/datasets/conll2003/conll2003.py , only I want to load the data from three local three-column tsv-files (id\ttokens\tpos_tags\n). I am using the most recent version of datasets. Thank you in advance!
Luisa
My script:
```
import datasets
import csv
logger = datasets.logging.get_logger(__name__)
class SampleConfig(datasets.BuilderConfig):
def __init__(self, **kwargs):
super(SampleConfig, self).__init__(**kwargs)
class Sample(datasets.GeneratorBasedBuilder):
BUILDER_CONFIGS = [
SampleConfig(name="conll2003", version=datasets.Version("1.0.0"), description="Conll2003 dataset"),
]
def _info(self):
return datasets.DatasetInfo(
description="Dataset with words and their POS-Tags",
features=datasets.Features(
{
"id": datasets.Value("string"),
"tokens": datasets.Sequence(datasets.Value("string")),
"pos_tags": datasets.Sequence(
datasets.features.ClassLabel(
names=[
"''",
",",
"-LRB-",
"-RRB-",
".",
":",
"CC",
"CD",
"DT",
"EX",
"FW",
"HYPH",
"IN",
"JJ",
"JJR",
"JJS",
"MD",
"NN",
"NNP",
"NNPS",
"NNS",
"PDT",
"POS",
"PRP",
"PRP$",
"RB",
"RBR",
"RBS",
"RP",
"TO",
"UH",
"VB",
"VBD",
"VBG",
"VBN",
"VBP",
"VBZ",
"WDT",
"WP",
"WRB",
"``"
]
)
),
}
),
supervised_keys=None,
homepage="https://catalog.ldc.upenn.edu/LDC2011T03",
citation="Weischedel, Ralph, et al. OntoNotes Release 4.0 LDC2011T03. Web Download. Philadelphia: Linguistic Data Consortium, 2011.",
)
def _split_generators(self, dl_manager):
loaded_files = dl_manager.download_and_extract(self.config.data_files)
return [
datasets.SplitGenerator(name=datasets.Split.TRAIN, gen_kwargs={"filepath": loaded_files["train"]}),
datasets.SplitGenerator(name=datasets.Split.TEST, gen_kwargs={"filepath": loaded_files["test"]}),
datasets.SplitGenerator(name=datasets.Split.VALIDATION, gen_kwargs={"filepath": loaded_files["val"]})
]
def _generate_examples(self, filepath):
logger.info("generating examples from = %s", filepath)
with open(filepath, encoding="cp1252") as f:
data = csv.reader(f, delimiter="\t")
ids = list()
tokens = list()
pos_tags = list()
for id_, line in enumerate(data):
#print(line)
if len(line) == 1:
if tokens:
yield id_, {"id": ids, "tokens": tokens, "pos_tags": pos_tags}
ids = list()
tokens = list()
pos_tags = list()
else:
ids.append(line[0])
tokens.append(line[1])
pos_tags.append(line[2])
# last example
yield id_, {"id": ids, "tokens": tokens, "pos_tags": pos_tags}
def main():
dataset = datasets.load_dataset(
"data_loading.py", data_files={
"train": "train.tsv",
"test": "test.tsv",
"val": "val.tsv"
}
)
#print(dataset)
if __name__=="__main__":
main()
```
| 230 | Windows Permission Error (most recent version of datasets)
Hi everyone,
Can anyone help me with why the dataset loading script below raises a Windows Permission Error? I stuck quite closely to https://github.com/huggingface/datasets/blob/master/datasets/conll2003/conll2003.py , only I want to load the data from three local three-column tsv-files (id\ttokens\tpos_tags\n). I am using the most recent version of datasets. Thank you in advance!
Luisa
My script:
```
import datasets
import csv
logger = datasets.logging.get_logger(__name__)
class SampleConfig(datasets.BuilderConfig):
def __init__(self, **kwargs):
super(SampleConfig, self).__init__(**kwargs)
class Sample(datasets.GeneratorBasedBuilder):
BUILDER_CONFIGS = [
SampleConfig(name="conll2003", version=datasets.Version("1.0.0"), description="Conll2003 dataset"),
]
def _info(self):
return datasets.DatasetInfo(
description="Dataset with words and their POS-Tags",
features=datasets.Features(
{
"id": datasets.Value("string"),
"tokens": datasets.Sequence(datasets.Value("string")),
"pos_tags": datasets.Sequence(
datasets.features.ClassLabel(
names=[
"''",
",",
"-LRB-",
"-RRB-",
".",
":",
"CC",
"CD",
"DT",
"EX",
"FW",
"HYPH",
"IN",
"JJ",
"JJR",
"JJS",
"MD",
"NN",
"NNP",
"NNPS",
"NNS",
"PDT",
"POS",
"PRP",
"PRP$",
"RB",
"RBR",
"RBS",
"RP",
"TO",
"UH",
"VB",
"VBD",
"VBG",
"VBN",
"VBP",
"VBZ",
"WDT",
"WP",
"WRB",
"``"
]
)
),
}
),
supervised_keys=None,
homepage="https://catalog.ldc.upenn.edu/LDC2011T03",
citation="Weischedel, Ralph, et al. OntoNotes Release 4.0 LDC2011T03. Web Download. Philadelphia: Linguistic Data Consortium, 2011.",
)
def _split_generators(self, dl_manager):
loaded_files = dl_manager.download_and_extract(self.config.data_files)
return [
datasets.SplitGenerator(name=datasets.Split.TRAIN, gen_kwargs={"filepath": loaded_files["train"]}),
datasets.SplitGenerator(name=datasets.Split.TEST, gen_kwargs={"filepath": loaded_files["test"]}),
datasets.SplitGenerator(name=datasets.Split.VALIDATION, gen_kwargs={"filepath": loaded_files["val"]})
]
def _generate_examples(self, filepath):
logger.info("generating examples from = %s", filepath)
with open(filepath, encoding="cp1252") as f:
data = csv.reader(f, delimiter="\t")
ids = list()
tokens = list()
pos_tags = list()
for id_, line in enumerate(data):
#print(line)
if len(line) == 1:
if tokens:
yield id_, {"id": ids, "tokens": tokens, "pos_tags": pos_tags}
ids = list()
tokens = list()
pos_tags = list()
else:
ids.append(line[0])
tokens.append(line[1])
pos_tags.append(line[2])
# last example
yield id_, {"id": ids, "tokens": tokens, "pos_tags": pos_tags}
def main():
dataset = datasets.load_dataset(
"data_loading.py", data_files={
"train": "train.tsv",
"test": "test.tsv",
"val": "val.tsv"
}
)
#print(dataset)
if __name__=="__main__":
main()
```
Hello @SBrandeis , this is it:
```
Traceback (most recent call last):
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\site-packages\datasets\builder.py", line 537, in incomplete_dir
yield tmp_dir
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\site-packages\datasets\builder.py", line 578, in download_and_prepare
self._download_and_prepare(
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\site-packages\datasets\builder.py", line 656, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\site-packages\datasets\builder.py", line 982, in _prepare_split
num_examples, num_bytes = writer.finalize()
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\site-packages\datasets\arrow_writer.py", line 297, in finalize
self.write_on_file()
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\site-packages\datasets\arrow_writer.py", line 230, in write_on_file
pa_array = pa.array(typed_sequence)
File "pyarrow\array.pxi", line 222, in pyarrow.lib.array
File "pyarrow\array.pxi", line 110, in pyarrow.lib._handle_arrow_array_protocol
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\site-packages\datasets\arrow_writer.py", line 97, in __arrow_array__
out = pa.array(self.data, type=type)
File "pyarrow\array.pxi", line 305, in pyarrow.lib.array
File "pyarrow\array.pxi", line 39, in pyarrow.lib._sequence_to_array
File "pyarrow\error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow\error.pxi", line 107, in pyarrow.lib.check_status
pyarrow.lib.ArrowTypeError: Expected bytes, got a 'list' object
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "C:/Users/Luisa/Documents/Uni/WS 2020,21/Neural Networks/Final_Project/NN_Project/data_loading.py", line 122, in <module>
main()
File "C:/Users/Luisa/Documents/Uni/WS 2020,21/Neural Networks/Final_Project/NN_Project/data_loading.py", line 111, in main
dataset = datasets.load_dataset(
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\site-packages\datasets\load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\site-packages\datasets\builder.py", line 586, in download_and_prepare
self._save_info()
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\contextlib.py", line 131, in __exit__
self.gen.throw(type, value, traceback)
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\site-packages\datasets\builder.py", line 543, in incomplete_dir
shutil.rmtree(tmp_dir)
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\shutil.py", line 740, in rmtree
return _rmtree_unsafe(path, onerror)
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\shutil.py", line 618, in _rmtree_unsafe
onerror(os.unlink, fullname, sys.exc_info())
File "C:\Users\Luisa\AppData\Local\Programs\Python\Python38\lib\shutil.py", line 616, in _rmtree_unsafe
os.unlink(fullname)
PermissionError: [WinError 32] Der Prozess kann nicht auf die Datei zugreifen, da sie von einem anderen Prozess verwendet wird: 'C:\\Users\\Luisa\\.cache\\huggingface\\datasets\\sample\\default-20ee7d51a6a9454f\\0.0.0\\5fc4c3a355ea77ab446bd31fca5082437600b8364d29b2b95264048bd1f398b1.incomplete\\sample-train.arrow'
Process finished with exit code 1
``` | [
-0.1825570911,
0.1823853403,
-0.0400311947,
0.2590482235,
0.0813781247,
0.1336375624,
0.4580341578,
0.0122546703,
0.1864631921,
0.0741284192,
-0.0634553432,
-0.0112266093,
-0.1087960601,
0.1572923511,
-0.0323619768,
-0.0282509699,
0.1453508139,
0.0480683856,
0.0319975466,
0.139645949,
-0.4052310884,
-0.007121121,
-0.2379361093,
0.1073664501,
-0.2231619209,
0.2783439457,
-0.0157265253,
0.3908287287,
-0.0338029079,
-0.2350180447,
0.2101651728,
0.3624859452,
0.6620944142,
0.2453529537,
-0.0001126285,
-0.0484501161,
0.0420434475,
-0.0201319195,
0.2068192661,
0.2423871309,
0.1341382414,
-0.04518703,
0.0041251788,
-0.1066692024,
-0.1698370129,
0.2000027448,
-0.0654299706,
-0.3538348079,
0.1139975935,
0.4263343811,
0.1871958673,
0.247462675,
-0.1806876957,
-0.0106539074,
0.5910393596,
0.0475985892,
-0.1261596382,
0.1927935481,
0.2900242507,
-0.4428054094,
0.2141413689,
0.1609823704,
-0.2268586606,
0.0855402499,
0.3818269372,
-0.1632637531,
-0.1299572885,
-0.283038944,
0.1584062576,
0.0000530392,
0.7270632982,
-0.1692434996,
-0.3009923398,
-0.0341044217,
0.1393089592,
-0.2671693265,
0.2275046855,
0.3365988135,
-0.050527174,
0.0753582194,
-0.2347000539,
-0.1671509296,
-0.443400681,
0.1358956397,
0.1775049269,
-0.2292980105,
-0.0849329084,
0.2297088653,
0.0906637385,
-0.1301722825,
0.3544328213,
0.1294435561,
0.0419616289,
0.2517597675,
-0.4671479762,
0.3360511959,
0.2092745006,
0.2980215251,
-0.0320270434,
0.2779500484,
-0.1361711621,
-0.2106274962,
0.2018986344,
0.1250197887,
0.1772743315,
-0.0542738587,
0.1519603282,
0.2411281615,
0.1375095099,
-0.02251116,
0.0402428508,
-0.1174408719,
-0.4900485575,
-0.6061115265,
0.1152088568,
0.2454816103,
0.3073365986,
-0.089267306,
-0.3613362312,
0.1164761037,
0.1181356385,
0.0680991709,
0.2068188041,
0.4097330272,
0.2663402855,
0.011945026,
0.3739323914,
0.2714996338,
-0.0628644973,
-0.0258000549,
-0.0862124264,
0.004246146,
-0.2458985001,
-0.0498456918,
0.4257870018,
-0.344258368,
-0.1134994254,
0.1173631102,
0.0353441276,
-0.1213496029,
0.0494541749,
-0.018771844,
0.1164599434,
0.438858062,
0.258418113,
0.0349972434,
0.1450433284,
-0.1851192862,
-0.0282775648,
0.2364420742,
-0.132414028,
-0.340477109,
-0.1883571893,
0.1011297554,
0.0170741994,
0.0499141589,
-0.2183059752,
-0.1330368519,
0.1228498742,
-0.018460894,
0.1442884654,
-0.1007024795,
-0.2660650909,
-0.3397949338,
-0.1015141979,
0.8314391375,
-0.5272638202,
0.1402403116,
-0.1147452071,
-0.304766953,
0.1072497815,
0.331196934,
-0.0764998272,
0.1113563403,
-0.2144830525,
0.1516457945,
-0.1357124448,
-0.368085146,
-0.1788970232,
0.3780058324,
-0.0177540891,
-0.1036801413,
0.2437340766,
-0.0390077457,
0.0444856919,
-0.2033563554,
-0.0898015723,
0.1341284364,
0.1384612024,
0.2605626285,
0.2249746919,
0.0075680506,
0.232209444,
0.312423408,
0.0553451143,
-0.0627512485,
0.2918674648,
-0.2186995149,
0.1895523965,
-0.3010882437,
0.2245456576,
0.4166682065,
0.1158196256,
0.3091973364,
0.0332214348,
-0.2322231084,
-0.4455741644,
0.2652017176,
0.3054820597,
-0.1239581332,
0.0469029248,
-0.2565839291,
-0.1502389908,
0.2109270543,
-0.3883989155,
0.1167707741,
0.0899114236,
0.2979707718,
-0.177402094,
-0.3208981156,
0.0345413946,
0.0563448817,
-0.2444297522,
-0.1111344323,
-0.1534329355,
0.03845869,
-0.3066702783,
0.0180421192,
0.2049439102,
-0.0148222595,
0.415769726,
-0.0743798465,
0.0026423864,
0.3591324985,
0.2803247273,
-0.0367781892,
0.0367961526,
0.0983091071,
-0.2261276245,
0.0771445036,
0.1272579283,
-0.0095818406,
0.0916863456,
-0.102523081,
-0.0255537909,
0.1265099198,
-0.2259501666,
0.0041123168,
-0.2879078686,
0.1314649731,
-0.0096178232,
0.0248836391,
-0.0246058516,
-0.2199263126,
0.3593811095,
0.5096368194,
-0.3285351992,
-0.0453587845,
-0.1026295051,
-0.0515074171,
0.2793928385,
-0.1505600214,
-0.1328615099,
0.0687072054,
0.0991908312,
0.2852438986,
0.1979035139,
0.2263827026,
0.4385740757,
0.0644595921,
-0.1653025001,
0.0529507995,
0.2032762915,
-0.0528066158,
0.1483860165,
-0.3353100419,
0.0793049783,
0.2603352368,
-0.299210012,
0.1275259107,
-0.3775517642,
-0.1403334588,
0.1440864801,
0.3369530141,
-0.5138479471,
-0.1326297224,
-0.0149591882,
-0.1334607005,
0.1105090231,
-0.3155809045,
-0.2831481993,
0.0130273448,
-0.2013535202,
0.162641421,
-0.2732631266,
-0.1256406903,
-0.4015874565,
0.0573597215,
0.2376945019,
-0.0031728656,
-0.0217247047,
0.0865614191,
-0.0238456167,
0.0146017903,
0.4932957292,
-0.28297472,
0.4370730221,
-0.1266709268,
0.3814795315,
-0.2898306549,
0.1060907543,
-0.2475937605,
-0.3344739377,
0.3203749657,
0.2462344468,
0.0983887985,
-0.0262449812,
-0.2058809102,
0.1195864901,
-0.1224582121,
-0.0811636224,
0.1755106896,
0.3042165041,
-0.5918186903,
-0.2506393492,
-0.1534151435,
-0.2042927295,
-0.3533948064,
0.2209897935,
0.1355350614,
0.3110540211,
0.2072858363,
-0.1218602806,
0.0530252792,
0.043456085,
0.3111527562,
-0.3197954595,
-0.0964616165,
0.0137316855,
-0.1502658129,
-0.5285491347,
0.2205064446,
0.3835148811,
-0.1217220724,
0.1091841757,
-0.1158390269,
0.125109762,
-0.2140982598,
0.2546712458,
-0.228287816,
0.1662581116,
0.1928659528,
0.0734915808,
0.0805567577,
-0.1694662571,
-0.0990941674,
-0.3450900316,
-0.2438754141,
-0.2646103203,
0.1542904377,
0.3330394924,
-0.131513983,
0.2149935961,
0.3426208198,
0.2264257967,
0.4461936951,
-0.1276844591,
0.4316770136,
0.2818518877,
-0.6437911391,
0.1521578133,
-0.18613033,
-0.05307265,
0.3601595163,
-0.0777109787,
0.0294725727,
-0.1574552804,
0.058306057,
-0.3378960192,
-0.2107928544,
-0.0072615365,
-0.2567558885,
0.2782018185,
-0.1479891986,
0.0072967792,
-0.2808843851,
-0.0628954396,
0.005472145,
0.5689313412,
0.1167745665,
-0.0616015047,
-0.3760633171,
0.0078707105,
-0.4856027961,
0.2696469426,
0.0624525286,
0.6091941595,
-0.1555263102,
0.0315031335,
0.1437780112,
0.1085257679,
0.9010461569,
-0.4391022623,
0.2763885856,
0.2481008172,
0.1166943014,
-0.2631775141,
-0.2115413994,
-0.2682220042,
0.0674349964,
-0.0131370639,
0.3030071557,
0.0118964575,
-0.3937036097,
0.0339969285,
-0.153051123,
-0.0794088542,
-0.4198120534,
-0.2535521388,
-0.1815235913,
-0.3771492243,
-0.104181312,
0.23979415,
0.1634427756,
-0.2563314438,
0.2497168928,
-0.0224106852,
0.2882218957,
0.1148119718,
-0.0406745709,
0.1016073227,
0.3072129786,
0.0786269903,
-0.0809461549,
0.1236321703,
0.2123339176,
0.5588173866,
-0.0261244364,
-0.3647989035,
-0.1107672825,
-0.0270075183,
0.4848946333,
0.0845979899,
-0.1533036828,
0.1014052853,
-0.2211104035,
-0.0586444661,
-0.3480155766,
0.0739531368,
0.2731053531,
-0.0515426248,
0.0595361367,
-0.2157611251,
0.3164066076,
0.1723265499,
-0.1943371892,
-0.0005693612,
0.1422990113,
-0.2947746515,
-0.0935067385,
0.0510760061,
0.5023057461,
-0.2940065563,
0.2797781825,
0.1955999881,
-0.1659104526,
-0.0711433366,
0.2135722637,
-0.0846961737,
-0.4017241597,
-0.3005034924,
-0.0134298494,
-0.1052670032,
-0.0054747174,
0.1585410684,
0.0849553198,
0.0400236696,
-0.0924143121,
0.3835963905,
0.0317606591,
0.0596931167,
-0.2020756453,
-0.2441046536,
0.1019106731,
0.1519687027,
-0.1361003816,
-0.0235723015,
-0.1311586946,
0.0135435546,
0.1534245759,
0.0239187609,
-0.3978717327,
0.2855560184,
-0.327996254,
0.010729772,
-0.1360234916,
0.0947397053,
0.5240967274,
0.4402703643,
-0.0064930753,
0.145454824,
-0.253116399,
-0.2666569054,
0.1540938616,
-0.2917835712,
-0.2915798128,
-0.0236649364,
0.2547367513,
0.0212812591,
-0.3019712567,
-0.1922041774,
-0.0331775397,
-0.243269071,
-0.0630601123,
0.4675464034,
0.1187869161,
-0.5070376396,
-0.1682939529,
-0.2320503443,
-0.0584002212,
0.0715505257,
0.1030867025,
0.1528619826,
-0.108543478,
0.061982438,
-0.2745188773,
-0.2699644566,
0.0699822605,
0.4720024765,
-0.275008291,
0.1021839082,
0.544580102,
0.4029548466,
-0.160260722,
-0.2423873544,
0.0846468583,
0.0800293684,
-0.2135887444,
0.0007552103,
0.3120194972,
0.0452675112,
0.4206766784,
0.0679823831,
0.2228616774,
-0.4020855725,
0.1897779852,
-0.4625520706,
-0.1102989763,
-0.0878792927,
-0.1858240962,
0.312094599,
0.0934093669,
-0.144258067,
0.0303128082,
-0.2334229499,
-0.2932747602,
0.1127860695,
-0.0025985059,
0.1187577322,
0.4522460699,
-0.2297088653,
-0.085651271,
-0.0219585076,
0.0636569858,
-0.1481773853,
-0.1781421304,
-0.2058388144,
0.0039552306,
0.1113950461,
0.1878647059,
-0.3387505114,
0.0858406574,
-0.5403543115,
-0.2182606459,
-0.1620230228,
0.2066287547,
0.2949579656,
-0.1138813123,
0.4738370776,
0.0064511574,
-0.0148760919,
0.0212340187,
0.1910280138,
-0.1915097386,
0.085947223,
-0.3789151311,
0.4132499099,
0.0258934665,
-0.085448429,
-0.3049194813,
0.2492911518,
0.2421406657,
0.1775054485,
0.1364677548,
-0.2938656211,
0.0452843755,
0.1257750988,
0.5298550725,
0.0940535814,
-0.3113822341,
0.030527601,
0.1404467821,
0.2037546486,
-0.3257119358,
-0.1571353972,
-0.2650842965,
-0.1184610054,
-0.0624470823,
0.1154166311,
0.011267364,
-0.3411979377,
-0.0892772675,
-0.1154887453,
0.2970601022,
0.2271779776,
-0.0939653441,
0.5441295505,
0.0009057375,
-0.0297026373,
0.3708810508,
0.5498603582,
0.0500367656,
0.4243816733,
0.1949298531,
-0.1030109748,
-0.1730054319,
0.0265197121,
-0.0050948379,
-0.7224724293,
0.1903757155,
-0.0895251706,
-0.0153278196,
0.0070984089,
-0.1846191734,
0.4962286949,
-0.1142301932,
-0.0175314061,
-0.3881717026,
0.1512206495,
-0.2028815299,
-0.1655405015,
-0.423861444,
0.0989476144,
-0.0877482966,
-0.0205381364,
-0.1252162457,
-0.246002242,
0.0037853292,
0.2284580171,
-0.032579381,
-0.2135649174,
0.3492715955,
0.2529511452,
-0.1378227323,
-0.3874363899,
-0.0780466571,
-0.0577865727,
0.120366253,
0.1329324841,
0.0222340617,
0.6245728731,
0.3318483531,
-0.0558945797,
-0.0126736993,
0.0129632708,
-0.0314593762,
-0.0356336199,
0.3866262138,
-0.2138805538,
0.3700493574,
0.3404142559,
0.0974965319,
-0.0588645488,
-0.0613858737,
0.039735008,
-0.1048274115,
-0.0849886015,
-0.0384140871,
-0.3763243258,
-0.2585603297,
-0.1624861807,
0.1023469418,
-0.0869154185,
-0.0223290734,
0.6900109053,
0.1503064334,
0.3419894278,
-0.3378277719,
0.0661960468,
0.0295752492,
0.4036604464,
0.4701581597,
0.0760131702,
-0.4037769139,
-0.0799949691,
-0.4968743622,
0.0621398464,
0.121179074,
-0.1317166239,
0.179227978,
-0.1361561269,
-0.0133228628,
-0.1985216886,
-0.0573431291,
0.0297258981,
0.0242386498,
0.3217082024,
-0.0086835288,
-0.1077248156,
0.265558064,
-0.0672237948,
0.142630592,
-0.5638712049,
0.0671391413,
-0.2215020061,
0.0575662628,
-0.4719405472,
0.0898200274,
0.123853825,
0.1396860182,
0.2431275547,
0.0155662708,
0.3771501482,
-0.0043459646,
0.1913797259,
-0.5739654899,
0.0678086728,
-0.1726516038,
0.143406868,
-0.1353252232,
-0.0251371469,
-0.2696663439,
0.0728009269,
-0.0983975381,
0.1188254878,
-0.0468874499,
0.1527617872,
-0.0512723923,
0.1282428652,
-0.1833433509,
-0.0416471846,
0.221635744,
-0.1437096447,
-0.1895446926,
0.3802647591,
-0.32337749,
-0.3723748326,
0.4683174789,
-0.2650414705,
-0.251288563,
0.0131053897,
0.3566475213,
-0.1201090291,
-0.1665671319,
-0.1439182609,
0.3625414073,
0.1724918336,
-0.0048149573,
-0.3380491436,
0.4713962674,
-0.203684479,
-0.1848140061,
0.0364144295,
0.423489362,
-0.0084359813,
-0.2079801559,
0.0341636352,
-0.1151267961
]
|
https://github.com/huggingface/datasets/issues/2000 | Windows Permission Error (most recent version of datasets) | Hi @itsLuisa, thanks for sharing the Traceback.
You are defining the "id" field as a `string` feature:
```python
class Sample(datasets.GeneratorBasedBuilder):
...
def _info(self):
return datasets.DatasetInfo(
features=datasets.Features(
{
"id": datasets.Value("string"),
# ^^ here
"tokens": datasets.Sequence(datasets.Value("string")),
"pos_tags": datasets.Sequence(datasets.features.ClassLabel(names=[...])),
[...]
```
But in the `_generate_examples`, the "id" field is a list:
```python
ids = list()
```
Changing:
```python
"id": datasets.Value("string"),
```
Into:
```python
"id": datasets.Sequence(datasets.Value("string")),
```
Should fix your issue.
Let me know if this helps! | Hi everyone,
Can anyone help me with why the dataset loading script below raises a Windows Permission Error? I stuck quite closely to https://github.com/huggingface/datasets/blob/master/datasets/conll2003/conll2003.py , only I want to load the data from three local three-column tsv-files (id\ttokens\tpos_tags\n). I am using the most recent version of datasets. Thank you in advance!
Luisa
My script:
```
import datasets
import csv
logger = datasets.logging.get_logger(__name__)
class SampleConfig(datasets.BuilderConfig):
def __init__(self, **kwargs):
super(SampleConfig, self).__init__(**kwargs)
class Sample(datasets.GeneratorBasedBuilder):
BUILDER_CONFIGS = [
SampleConfig(name="conll2003", version=datasets.Version("1.0.0"), description="Conll2003 dataset"),
]
def _info(self):
return datasets.DatasetInfo(
description="Dataset with words and their POS-Tags",
features=datasets.Features(
{
"id": datasets.Value("string"),
"tokens": datasets.Sequence(datasets.Value("string")),
"pos_tags": datasets.Sequence(
datasets.features.ClassLabel(
names=[
"''",
",",
"-LRB-",
"-RRB-",
".",
":",
"CC",
"CD",
"DT",
"EX",
"FW",
"HYPH",
"IN",
"JJ",
"JJR",
"JJS",
"MD",
"NN",
"NNP",
"NNPS",
"NNS",
"PDT",
"POS",
"PRP",
"PRP$",
"RB",
"RBR",
"RBS",
"RP",
"TO",
"UH",
"VB",
"VBD",
"VBG",
"VBN",
"VBP",
"VBZ",
"WDT",
"WP",
"WRB",
"``"
]
)
),
}
),
supervised_keys=None,
homepage="https://catalog.ldc.upenn.edu/LDC2011T03",
citation="Weischedel, Ralph, et al. OntoNotes Release 4.0 LDC2011T03. Web Download. Philadelphia: Linguistic Data Consortium, 2011.",
)
def _split_generators(self, dl_manager):
loaded_files = dl_manager.download_and_extract(self.config.data_files)
return [
datasets.SplitGenerator(name=datasets.Split.TRAIN, gen_kwargs={"filepath": loaded_files["train"]}),
datasets.SplitGenerator(name=datasets.Split.TEST, gen_kwargs={"filepath": loaded_files["test"]}),
datasets.SplitGenerator(name=datasets.Split.VALIDATION, gen_kwargs={"filepath": loaded_files["val"]})
]
def _generate_examples(self, filepath):
logger.info("generating examples from = %s", filepath)
with open(filepath, encoding="cp1252") as f:
data = csv.reader(f, delimiter="\t")
ids = list()
tokens = list()
pos_tags = list()
for id_, line in enumerate(data):
#print(line)
if len(line) == 1:
if tokens:
yield id_, {"id": ids, "tokens": tokens, "pos_tags": pos_tags}
ids = list()
tokens = list()
pos_tags = list()
else:
ids.append(line[0])
tokens.append(line[1])
pos_tags.append(line[2])
# last example
yield id_, {"id": ids, "tokens": tokens, "pos_tags": pos_tags}
def main():
dataset = datasets.load_dataset(
"data_loading.py", data_files={
"train": "train.tsv",
"test": "test.tsv",
"val": "val.tsv"
}
)
#print(dataset)
if __name__=="__main__":
main()
```
| 73 | Windows Permission Error (most recent version of datasets)
Hi everyone,
Can anyone help me with why the dataset loading script below raises a Windows Permission Error? I stuck quite closely to https://github.com/huggingface/datasets/blob/master/datasets/conll2003/conll2003.py , only I want to load the data from three local three-column tsv-files (id\ttokens\tpos_tags\n). I am using the most recent version of datasets. Thank you in advance!
Luisa
My script:
```
import datasets
import csv
logger = datasets.logging.get_logger(__name__)
class SampleConfig(datasets.BuilderConfig):
def __init__(self, **kwargs):
super(SampleConfig, self).__init__(**kwargs)
class Sample(datasets.GeneratorBasedBuilder):
BUILDER_CONFIGS = [
SampleConfig(name="conll2003", version=datasets.Version("1.0.0"), description="Conll2003 dataset"),
]
def _info(self):
return datasets.DatasetInfo(
description="Dataset with words and their POS-Tags",
features=datasets.Features(
{
"id": datasets.Value("string"),
"tokens": datasets.Sequence(datasets.Value("string")),
"pos_tags": datasets.Sequence(
datasets.features.ClassLabel(
names=[
"''",
",",
"-LRB-",
"-RRB-",
".",
":",
"CC",
"CD",
"DT",
"EX",
"FW",
"HYPH",
"IN",
"JJ",
"JJR",
"JJS",
"MD",
"NN",
"NNP",
"NNPS",
"NNS",
"PDT",
"POS",
"PRP",
"PRP$",
"RB",
"RBR",
"RBS",
"RP",
"TO",
"UH",
"VB",
"VBD",
"VBG",
"VBN",
"VBP",
"VBZ",
"WDT",
"WP",
"WRB",
"``"
]
)
),
}
),
supervised_keys=None,
homepage="https://catalog.ldc.upenn.edu/LDC2011T03",
citation="Weischedel, Ralph, et al. OntoNotes Release 4.0 LDC2011T03. Web Download. Philadelphia: Linguistic Data Consortium, 2011.",
)
def _split_generators(self, dl_manager):
loaded_files = dl_manager.download_and_extract(self.config.data_files)
return [
datasets.SplitGenerator(name=datasets.Split.TRAIN, gen_kwargs={"filepath": loaded_files["train"]}),
datasets.SplitGenerator(name=datasets.Split.TEST, gen_kwargs={"filepath": loaded_files["test"]}),
datasets.SplitGenerator(name=datasets.Split.VALIDATION, gen_kwargs={"filepath": loaded_files["val"]})
]
def _generate_examples(self, filepath):
logger.info("generating examples from = %s", filepath)
with open(filepath, encoding="cp1252") as f:
data = csv.reader(f, delimiter="\t")
ids = list()
tokens = list()
pos_tags = list()
for id_, line in enumerate(data):
#print(line)
if len(line) == 1:
if tokens:
yield id_, {"id": ids, "tokens": tokens, "pos_tags": pos_tags}
ids = list()
tokens = list()
pos_tags = list()
else:
ids.append(line[0])
tokens.append(line[1])
pos_tags.append(line[2])
# last example
yield id_, {"id": ids, "tokens": tokens, "pos_tags": pos_tags}
def main():
dataset = datasets.load_dataset(
"data_loading.py", data_files={
"train": "train.tsv",
"test": "test.tsv",
"val": "val.tsv"
}
)
#print(dataset)
if __name__=="__main__":
main()
```
Hi @itsLuisa, thanks for sharing the Traceback.
You are defining the "id" field as a `string` feature:
```python
class Sample(datasets.GeneratorBasedBuilder):
...
def _info(self):
return datasets.DatasetInfo(
features=datasets.Features(
{
"id": datasets.Value("string"),
# ^^ here
"tokens": datasets.Sequence(datasets.Value("string")),
"pos_tags": datasets.Sequence(datasets.features.ClassLabel(names=[...])),
[...]
```
But in the `_generate_examples`, the "id" field is a list:
```python
ids = list()
```
Changing:
```python
"id": datasets.Value("string"),
```
Into:
```python
"id": datasets.Sequence(datasets.Value("string")),
```
Should fix your issue.
Let me know if this helps! | [
-0.1825570911,
0.1823853403,
-0.0400311947,
0.2590482235,
0.0813781247,
0.1336375624,
0.4580341578,
0.0122546703,
0.1864631921,
0.0741284192,
-0.0634553432,
-0.0112266093,
-0.1087960601,
0.1572923511,
-0.0323619768,
-0.0282509699,
0.1453508139,
0.0480683856,
0.0319975466,
0.139645949,
-0.4052310884,
-0.007121121,
-0.2379361093,
0.1073664501,
-0.2231619209,
0.2783439457,
-0.0157265253,
0.3908287287,
-0.0338029079,
-0.2350180447,
0.2101651728,
0.3624859452,
0.6620944142,
0.2453529537,
-0.0001126285,
-0.0484501161,
0.0420434475,
-0.0201319195,
0.2068192661,
0.2423871309,
0.1341382414,
-0.04518703,
0.0041251788,
-0.1066692024,
-0.1698370129,
0.2000027448,
-0.0654299706,
-0.3538348079,
0.1139975935,
0.4263343811,
0.1871958673,
0.247462675,
-0.1806876957,
-0.0106539074,
0.5910393596,
0.0475985892,
-0.1261596382,
0.1927935481,
0.2900242507,
-0.4428054094,
0.2141413689,
0.1609823704,
-0.2268586606,
0.0855402499,
0.3818269372,
-0.1632637531,
-0.1299572885,
-0.283038944,
0.1584062576,
0.0000530392,
0.7270632982,
-0.1692434996,
-0.3009923398,
-0.0341044217,
0.1393089592,
-0.2671693265,
0.2275046855,
0.3365988135,
-0.050527174,
0.0753582194,
-0.2347000539,
-0.1671509296,
-0.443400681,
0.1358956397,
0.1775049269,
-0.2292980105,
-0.0849329084,
0.2297088653,
0.0906637385,
-0.1301722825,
0.3544328213,
0.1294435561,
0.0419616289,
0.2517597675,
-0.4671479762,
0.3360511959,
0.2092745006,
0.2980215251,
-0.0320270434,
0.2779500484,
-0.1361711621,
-0.2106274962,
0.2018986344,
0.1250197887,
0.1772743315,
-0.0542738587,
0.1519603282,
0.2411281615,
0.1375095099,
-0.02251116,
0.0402428508,
-0.1174408719,
-0.4900485575,
-0.6061115265,
0.1152088568,
0.2454816103,
0.3073365986,
-0.089267306,
-0.3613362312,
0.1164761037,
0.1181356385,
0.0680991709,
0.2068188041,
0.4097330272,
0.2663402855,
0.011945026,
0.3739323914,
0.2714996338,
-0.0628644973,
-0.0258000549,
-0.0862124264,
0.004246146,
-0.2458985001,
-0.0498456918,
0.4257870018,
-0.344258368,
-0.1134994254,
0.1173631102,
0.0353441276,
-0.1213496029,
0.0494541749,
-0.018771844,
0.1164599434,
0.438858062,
0.258418113,
0.0349972434,
0.1450433284,
-0.1851192862,
-0.0282775648,
0.2364420742,
-0.132414028,
-0.340477109,
-0.1883571893,
0.1011297554,
0.0170741994,
0.0499141589,
-0.2183059752,
-0.1330368519,
0.1228498742,
-0.018460894,
0.1442884654,
-0.1007024795,
-0.2660650909,
-0.3397949338,
-0.1015141979,
0.8314391375,
-0.5272638202,
0.1402403116,
-0.1147452071,
-0.304766953,
0.1072497815,
0.331196934,
-0.0764998272,
0.1113563403,
-0.2144830525,
0.1516457945,
-0.1357124448,
-0.368085146,
-0.1788970232,
0.3780058324,
-0.0177540891,
-0.1036801413,
0.2437340766,
-0.0390077457,
0.0444856919,
-0.2033563554,
-0.0898015723,
0.1341284364,
0.1384612024,
0.2605626285,
0.2249746919,
0.0075680506,
0.232209444,
0.312423408,
0.0553451143,
-0.0627512485,
0.2918674648,
-0.2186995149,
0.1895523965,
-0.3010882437,
0.2245456576,
0.4166682065,
0.1158196256,
0.3091973364,
0.0332214348,
-0.2322231084,
-0.4455741644,
0.2652017176,
0.3054820597,
-0.1239581332,
0.0469029248,
-0.2565839291,
-0.1502389908,
0.2109270543,
-0.3883989155,
0.1167707741,
0.0899114236,
0.2979707718,
-0.177402094,
-0.3208981156,
0.0345413946,
0.0563448817,
-0.2444297522,
-0.1111344323,
-0.1534329355,
0.03845869,
-0.3066702783,
0.0180421192,
0.2049439102,
-0.0148222595,
0.415769726,
-0.0743798465,
0.0026423864,
0.3591324985,
0.2803247273,
-0.0367781892,
0.0367961526,
0.0983091071,
-0.2261276245,
0.0771445036,
0.1272579283,
-0.0095818406,
0.0916863456,
-0.102523081,
-0.0255537909,
0.1265099198,
-0.2259501666,
0.0041123168,
-0.2879078686,
0.1314649731,
-0.0096178232,
0.0248836391,
-0.0246058516,
-0.2199263126,
0.3593811095,
0.5096368194,
-0.3285351992,
-0.0453587845,
-0.1026295051,
-0.0515074171,
0.2793928385,
-0.1505600214,
-0.1328615099,
0.0687072054,
0.0991908312,
0.2852438986,
0.1979035139,
0.2263827026,
0.4385740757,
0.0644595921,
-0.1653025001,
0.0529507995,
0.2032762915,
-0.0528066158,
0.1483860165,
-0.3353100419,
0.0793049783,
0.2603352368,
-0.299210012,
0.1275259107,
-0.3775517642,
-0.1403334588,
0.1440864801,
0.3369530141,
-0.5138479471,
-0.1326297224,
-0.0149591882,
-0.1334607005,
0.1105090231,
-0.3155809045,
-0.2831481993,
0.0130273448,
-0.2013535202,
0.162641421,
-0.2732631266,
-0.1256406903,
-0.4015874565,
0.0573597215,
0.2376945019,
-0.0031728656,
-0.0217247047,
0.0865614191,
-0.0238456167,
0.0146017903,
0.4932957292,
-0.28297472,
0.4370730221,
-0.1266709268,
0.3814795315,
-0.2898306549,
0.1060907543,
-0.2475937605,
-0.3344739377,
0.3203749657,
0.2462344468,
0.0983887985,
-0.0262449812,
-0.2058809102,
0.1195864901,
-0.1224582121,
-0.0811636224,
0.1755106896,
0.3042165041,
-0.5918186903,
-0.2506393492,
-0.1534151435,
-0.2042927295,
-0.3533948064,
0.2209897935,
0.1355350614,
0.3110540211,
0.2072858363,
-0.1218602806,
0.0530252792,
0.043456085,
0.3111527562,
-0.3197954595,
-0.0964616165,
0.0137316855,
-0.1502658129,
-0.5285491347,
0.2205064446,
0.3835148811,
-0.1217220724,
0.1091841757,
-0.1158390269,
0.125109762,
-0.2140982598,
0.2546712458,
-0.228287816,
0.1662581116,
0.1928659528,
0.0734915808,
0.0805567577,
-0.1694662571,
-0.0990941674,
-0.3450900316,
-0.2438754141,
-0.2646103203,
0.1542904377,
0.3330394924,
-0.131513983,
0.2149935961,
0.3426208198,
0.2264257967,
0.4461936951,
-0.1276844591,
0.4316770136,
0.2818518877,
-0.6437911391,
0.1521578133,
-0.18613033,
-0.05307265,
0.3601595163,
-0.0777109787,
0.0294725727,
-0.1574552804,
0.058306057,
-0.3378960192,
-0.2107928544,
-0.0072615365,
-0.2567558885,
0.2782018185,
-0.1479891986,
0.0072967792,
-0.2808843851,
-0.0628954396,
0.005472145,
0.5689313412,
0.1167745665,
-0.0616015047,
-0.3760633171,
0.0078707105,
-0.4856027961,
0.2696469426,
0.0624525286,
0.6091941595,
-0.1555263102,
0.0315031335,
0.1437780112,
0.1085257679,
0.9010461569,
-0.4391022623,
0.2763885856,
0.2481008172,
0.1166943014,
-0.2631775141,
-0.2115413994,
-0.2682220042,
0.0674349964,
-0.0131370639,
0.3030071557,
0.0118964575,
-0.3937036097,
0.0339969285,
-0.153051123,
-0.0794088542,
-0.4198120534,
-0.2535521388,
-0.1815235913,
-0.3771492243,
-0.104181312,
0.23979415,
0.1634427756,
-0.2563314438,
0.2497168928,
-0.0224106852,
0.2882218957,
0.1148119718,
-0.0406745709,
0.1016073227,
0.3072129786,
0.0786269903,
-0.0809461549,
0.1236321703,
0.2123339176,
0.5588173866,
-0.0261244364,
-0.3647989035,
-0.1107672825,
-0.0270075183,
0.4848946333,
0.0845979899,
-0.1533036828,
0.1014052853,
-0.2211104035,
-0.0586444661,
-0.3480155766,
0.0739531368,
0.2731053531,
-0.0515426248,
0.0595361367,
-0.2157611251,
0.3164066076,
0.1723265499,
-0.1943371892,
-0.0005693612,
0.1422990113,
-0.2947746515,
-0.0935067385,
0.0510760061,
0.5023057461,
-0.2940065563,
0.2797781825,
0.1955999881,
-0.1659104526,
-0.0711433366,
0.2135722637,
-0.0846961737,
-0.4017241597,
-0.3005034924,
-0.0134298494,
-0.1052670032,
-0.0054747174,
0.1585410684,
0.0849553198,
0.0400236696,
-0.0924143121,
0.3835963905,
0.0317606591,
0.0596931167,
-0.2020756453,
-0.2441046536,
0.1019106731,
0.1519687027,
-0.1361003816,
-0.0235723015,
-0.1311586946,
0.0135435546,
0.1534245759,
0.0239187609,
-0.3978717327,
0.2855560184,
-0.327996254,
0.010729772,
-0.1360234916,
0.0947397053,
0.5240967274,
0.4402703643,
-0.0064930753,
0.145454824,
-0.253116399,
-0.2666569054,
0.1540938616,
-0.2917835712,
-0.2915798128,
-0.0236649364,
0.2547367513,
0.0212812591,
-0.3019712567,
-0.1922041774,
-0.0331775397,
-0.243269071,
-0.0630601123,
0.4675464034,
0.1187869161,
-0.5070376396,
-0.1682939529,
-0.2320503443,
-0.0584002212,
0.0715505257,
0.1030867025,
0.1528619826,
-0.108543478,
0.061982438,
-0.2745188773,
-0.2699644566,
0.0699822605,
0.4720024765,
-0.275008291,
0.1021839082,
0.544580102,
0.4029548466,
-0.160260722,
-0.2423873544,
0.0846468583,
0.0800293684,
-0.2135887444,
0.0007552103,
0.3120194972,
0.0452675112,
0.4206766784,
0.0679823831,
0.2228616774,
-0.4020855725,
0.1897779852,
-0.4625520706,
-0.1102989763,
-0.0878792927,
-0.1858240962,
0.312094599,
0.0934093669,
-0.144258067,
0.0303128082,
-0.2334229499,
-0.2932747602,
0.1127860695,
-0.0025985059,
0.1187577322,
0.4522460699,
-0.2297088653,
-0.085651271,
-0.0219585076,
0.0636569858,
-0.1481773853,
-0.1781421304,
-0.2058388144,
0.0039552306,
0.1113950461,
0.1878647059,
-0.3387505114,
0.0858406574,
-0.5403543115,
-0.2182606459,
-0.1620230228,
0.2066287547,
0.2949579656,
-0.1138813123,
0.4738370776,
0.0064511574,
-0.0148760919,
0.0212340187,
0.1910280138,
-0.1915097386,
0.085947223,
-0.3789151311,
0.4132499099,
0.0258934665,
-0.085448429,
-0.3049194813,
0.2492911518,
0.2421406657,
0.1775054485,
0.1364677548,
-0.2938656211,
0.0452843755,
0.1257750988,
0.5298550725,
0.0940535814,
-0.3113822341,
0.030527601,
0.1404467821,
0.2037546486,
-0.3257119358,
-0.1571353972,
-0.2650842965,
-0.1184610054,
-0.0624470823,
0.1154166311,
0.011267364,
-0.3411979377,
-0.0892772675,
-0.1154887453,
0.2970601022,
0.2271779776,
-0.0939653441,
0.5441295505,
0.0009057375,
-0.0297026373,
0.3708810508,
0.5498603582,
0.0500367656,
0.4243816733,
0.1949298531,
-0.1030109748,
-0.1730054319,
0.0265197121,
-0.0050948379,
-0.7224724293,
0.1903757155,
-0.0895251706,
-0.0153278196,
0.0070984089,
-0.1846191734,
0.4962286949,
-0.1142301932,
-0.0175314061,
-0.3881717026,
0.1512206495,
-0.2028815299,
-0.1655405015,
-0.423861444,
0.0989476144,
-0.0877482966,
-0.0205381364,
-0.1252162457,
-0.246002242,
0.0037853292,
0.2284580171,
-0.032579381,
-0.2135649174,
0.3492715955,
0.2529511452,
-0.1378227323,
-0.3874363899,
-0.0780466571,
-0.0577865727,
0.120366253,
0.1329324841,
0.0222340617,
0.6245728731,
0.3318483531,
-0.0558945797,
-0.0126736993,
0.0129632708,
-0.0314593762,
-0.0356336199,
0.3866262138,
-0.2138805538,
0.3700493574,
0.3404142559,
0.0974965319,
-0.0588645488,
-0.0613858737,
0.039735008,
-0.1048274115,
-0.0849886015,
-0.0384140871,
-0.3763243258,
-0.2585603297,
-0.1624861807,
0.1023469418,
-0.0869154185,
-0.0223290734,
0.6900109053,
0.1503064334,
0.3419894278,
-0.3378277719,
0.0661960468,
0.0295752492,
0.4036604464,
0.4701581597,
0.0760131702,
-0.4037769139,
-0.0799949691,
-0.4968743622,
0.0621398464,
0.121179074,
-0.1317166239,
0.179227978,
-0.1361561269,
-0.0133228628,
-0.1985216886,
-0.0573431291,
0.0297258981,
0.0242386498,
0.3217082024,
-0.0086835288,
-0.1077248156,
0.265558064,
-0.0672237948,
0.142630592,
-0.5638712049,
0.0671391413,
-0.2215020061,
0.0575662628,
-0.4719405472,
0.0898200274,
0.123853825,
0.1396860182,
0.2431275547,
0.0155662708,
0.3771501482,
-0.0043459646,
0.1913797259,
-0.5739654899,
0.0678086728,
-0.1726516038,
0.143406868,
-0.1353252232,
-0.0251371469,
-0.2696663439,
0.0728009269,
-0.0983975381,
0.1188254878,
-0.0468874499,
0.1527617872,
-0.0512723923,
0.1282428652,
-0.1833433509,
-0.0416471846,
0.221635744,
-0.1437096447,
-0.1895446926,
0.3802647591,
-0.32337749,
-0.3723748326,
0.4683174789,
-0.2650414705,
-0.251288563,
0.0131053897,
0.3566475213,
-0.1201090291,
-0.1665671319,
-0.1439182609,
0.3625414073,
0.1724918336,
-0.0048149573,
-0.3380491436,
0.4713962674,
-0.203684479,
-0.1848140061,
0.0364144295,
0.423489362,
-0.0084359813,
-0.2079801559,
0.0341636352,
-0.1151267961
]
|
https://github.com/huggingface/datasets/issues/1996 | Error when exploring `arabic_speech_corpus` | Actually soundfile is not a dependency of this dataset.
The error comes from a bug that was fixed in this commit: https://github.com/huggingface/datasets/pull/1767/commits/c304e63629f4453367de2fd42883a78768055532
Basically the library used to consider the `import soundfile` in the docstring as a dependency, while it's just here as a code example.
Updating the viewer to the latest version of `datasets` should fix this issue
| Navigate to https://huggingface.co/datasets/viewer/?dataset=arabic_speech_corpus
Error:
```
ImportError: To be able to use this dataset, you need to install the following dependencies['soundfile'] using 'pip install soundfile' for instance'
Traceback:
File "/home/sasha/.local/share/virtualenvs/lib-ogGKnCK_/lib/python3.7/site-packages/streamlit/script_runner.py", line 332, in _run_script
exec(code, module.__dict__)
File "/home/sasha/nlp-viewer/run.py", line 233, in <module>
configs = get_confs(option)
File "/home/sasha/.local/share/virtualenvs/lib-ogGKnCK_/lib/python3.7/site-packages/streamlit/caching.py", line 604, in wrapped_func
return get_or_create_cached_value()
File "/home/sasha/.local/share/virtualenvs/lib-ogGKnCK_/lib/python3.7/site-packages/streamlit/caching.py", line 588, in get_or_create_cached_value
return_value = func(*args, **kwargs)
File "/home/sasha/nlp-viewer/run.py", line 145, in get_confs
module_path = nlp.load.prepare_module(path, dataset=True
File "/home/sasha/.local/share/virtualenvs/lib-ogGKnCK_/lib/python3.7/site-packages/datasets/load.py", line 342, in prepare_module
f"To be able to use this {module_type}, you need to install the following dependencies"
``` | 58 | Error when exploring `arabic_speech_corpus`
Navigate to https://huggingface.co/datasets/viewer/?dataset=arabic_speech_corpus
Error:
```
ImportError: To be able to use this dataset, you need to install the following dependencies['soundfile'] using 'pip install soundfile' for instance'
Traceback:
File "/home/sasha/.local/share/virtualenvs/lib-ogGKnCK_/lib/python3.7/site-packages/streamlit/script_runner.py", line 332, in _run_script
exec(code, module.__dict__)
File "/home/sasha/nlp-viewer/run.py", line 233, in <module>
configs = get_confs(option)
File "/home/sasha/.local/share/virtualenvs/lib-ogGKnCK_/lib/python3.7/site-packages/streamlit/caching.py", line 604, in wrapped_func
return get_or_create_cached_value()
File "/home/sasha/.local/share/virtualenvs/lib-ogGKnCK_/lib/python3.7/site-packages/streamlit/caching.py", line 588, in get_or_create_cached_value
return_value = func(*args, **kwargs)
File "/home/sasha/nlp-viewer/run.py", line 145, in get_confs
module_path = nlp.load.prepare_module(path, dataset=True
File "/home/sasha/.local/share/virtualenvs/lib-ogGKnCK_/lib/python3.7/site-packages/datasets/load.py", line 342, in prepare_module
f"To be able to use this {module_type}, you need to install the following dependencies"
```
Actually soundfile is not a dependency of this dataset.
The error comes from a bug that was fixed in this commit: https://github.com/huggingface/datasets/pull/1767/commits/c304e63629f4453367de2fd42883a78768055532
Basically the library used to consider the `import soundfile` in the docstring as a dependency, while it's just here as a code example.
Updating the viewer to the latest version of `datasets` should fix this issue
| [
-0.259124577,
-0.1135888472,
-0.0363893025,
0.2228231132,
0.041789569,
0.0414842777,
0.0535817631,
0.3140556514,
-0.1731434911,
0.0504595041,
-0.2981741726,
0.0969086662,
-0.1112823859,
-0.0406438522,
0.1065410078,
-0.3713723123,
0.0633600503,
0.203241244,
0.0054918919,
-0.0132910395,
-0.0697459206,
0.4542388916,
-0.3286395073,
-0.0139065282,
-0.0968817174,
-0.2608727217,
-0.0601529554,
-0.1154938787,
-0.2918643653,
-0.4660019875,
0.1471933275,
-0.0764239356,
0.0929138437,
0.3895767629,
-0.0001143709,
-0.0865352228,
0.36810413,
-0.1396298856,
-0.2088978142,
-0.4318642914,
0.1541050225,
-0.1369882822,
-0.0995632261,
-0.1122165024,
-0.0662244856,
-0.1979068369,
0.0398826078,
-0.3098417222,
0.291513443,
0.3186622262,
0.2341215611,
0.1870320886,
0.2347774655,
-0.041816365,
0.2049754411,
-0.0016586902,
-0.1434953362,
0.2094646841,
0.1850298196,
0.0741268694,
-0.1772948354,
0.5136581063,
-0.3165773451,
-0.0839221627,
0.0919753537,
-0.1553822011,
-0.0313265137,
-0.4021749198,
0.1798989922,
-0.0613103621,
0.4357356131,
-0.3616387546,
-0.242231518,
-0.2717442811,
0.1527152956,
-0.2331832051,
0.2647303641,
0.3108145595,
-0.2944635749,
0.1316216737,
0.0926429927,
-0.2117517292,
-0.0141711524,
0.1655192822,
0.0573513508,
0.2916788459,
-0.299402684,
-0.1460859627,
0.3502129614,
-0.1967081279,
-0.2485626191,
0.047421176,
-0.0852020308,
0.2425547242,
-0.065177083,
-0.0221834425,
0.163206622,
0.1580074728,
-0.0387696289,
0.0165189821,
-0.1195857674,
0.2261200696,
-0.1795152426,
0.2216258645,
-0.0141471755,
0.1789913327,
0.2417351753,
-0.020095503,
0.2245755643,
0.4123168588,
0.0120384302,
0.0000340128,
-0.0760708824,
-0.1948830932,
-0.4148891866,
-0.0548376478,
0.5135284662,
-0.2739717066,
-0.155549556,
0.0554306433,
-0.078681618,
-0.220929727,
0.096221149,
0.4137219787,
-0.1246659383,
-0.0105804559,
0.1273990721,
0.3244033754,
-0.2293331772,
-0.2025601119,
0.0115853259,
0.2179546505,
-0.0469129942,
0.1443845928,
0.2509666681,
-0.4630836844,
0.5074710846,
0.0049602585,
0.1702277809,
0.1916876733,
-0.0764002651,
-0.0905367061,
-0.0938393921,
0.3047252893,
-0.0437417068,
0.1594018936,
0.2130077183,
-0.0378161073,
-0.2543686926,
0.0117811719,
0.031125471,
-0.217360422,
-0.0682516918,
0.1823176146,
-0.3190979362,
-0.0554398,
-0.1916363984,
0.2330010235,
0.1791095287,
-0.4966381788,
-0.0456907973,
0.0339264758,
-0.531935811,
-0.0135487122,
0.0597185642,
0.4067558348,
-0.0727341622,
-0.2927670479,
-0.0841919705,
0.0253015533,
0.1555550694,
0.1164547056,
-0.0388879515,
0.0291496217,
-0.2782106698,
0.3276976943,
0.3454293609,
-0.5265834332,
-0.4309079349,
0.1337178349,
0.1185406074,
0.2625015974,
0.3312529624,
-0.2331043929,
0.2469704002,
-0.2004734725,
0.1713262498,
0.3000825942,
0.1903236806,
-0.1005734652,
-0.1891233772,
-0.1464090943,
-0.0314407088,
0.0946112573,
-0.1408828199,
0.1675129682,
-0.001527737,
-0.0004478744,
0.1974836737,
0.0762269273,
0.0679527298,
0.2516247034,
0.2334306389,
0.0558911227,
0.0565041602,
-0.4098464847,
0.0838469118,
0.0377535895,
-0.3253623247,
0.425362289,
-0.4172177613,
-0.135989055,
-0.2352314591,
-0.0995791778,
-0.241177246,
-0.1433662325,
0.1581528783,
0.2674128115,
0.1796350032,
0.3149421215,
-0.2450620979,
-0.0018465914,
0.1778331846,
-0.0270124339,
-0.6278398037,
0.08613047,
-0.2138070017,
-0.1113750339,
0.3707225621,
0.2790820301,
0.1563702524,
-0.1181211248,
-0.0805432424,
0.221309334,
-0.0635786355,
0.336964637,
-0.4050097466,
0.1184294373,
0.1225037351,
-0.3153544664,
0.3848434985,
0.1509908736,
0.124534443,
-0.0551245958,
0.3454687297,
0.1909190416,
0.2136060148,
0.1452515572,
0.1701562107,
0.0841466486,
0.448058933,
0.1295616925,
-0.224626258,
-0.3109560013,
0.4079636335,
-0.2685557306,
0.5072847009,
0.0207664818,
-0.3180300891,
0.007370377,
0.5209944248,
-0.0545144118,
0.184432596,
0.1243081465,
-0.3222735524,
-0.1683202386,
0.256219089,
0.1419637799,
0.4471821487,
0.1286049336,
0.077922605,
0.1960663795,
0.0627728924,
-0.3435525298,
0.2704866827,
0.0305355731,
0.0706947446,
0.2770345807,
0.0956615359,
0.0486450605,
-0.5301123261,
-0.0327140093,
-0.1379945129,
0.1653426439,
-0.1218274832,
0.0161712337,
-0.3099751174,
-0.5472097993,
-0.1764029562,
-0.2263454348,
-0.36137712,
-0.1843631268,
0.0760280937,
0.258405596,
0.0806571618,
0.3466959894,
0.1766172349,
0.0651237741,
-0.155027777,
-0.1836979836,
-0.2633356154,
-0.0257067755,
-0.1293577403,
0.0739427432,
0.3326383233,
0.2319952548,
0.2194442153,
-0.124803111,
-0.0389510244,
-0.2586820722,
-0.1783269197,
0.2145291269,
-0.2075853795,
0.1726352274,
0.1471444666,
0.140346095,
-0.0251220819,
-0.3683166802,
0.2150207907,
0.0055193133,
-0.08204218,
0.1785070002,
-0.1032172665,
0.0165979303,
-0.1582723409,
-0.2487446815,
-0.2342049628,
-0.5632392168,
0.0237039234,
0.0359938778,
0.1542962193,
0.6405218244,
-0.0233093966,
0.2233795226,
-0.1866189688,
0.4464222789,
-0.1898086965,
-0.2654584944,
0.3701419234,
-0.3133113086,
-0.385119319,
0.0832384378,
0.0741172358,
0.5423163772,
-0.1641804278,
-0.4779160619,
-0.0479014739,
-0.2519606054,
-0.0908006057,
-0.0273993164,
0.1235231608,
0.3727433383,
0.1244203597,
-0.0558640882,
-0.1544631869,
-0.1127558127,
-0.2120383531,
0.0303631965,
0.1103433967,
0.117339462,
0.3938212097,
0.0364327542,
0.5581878424,
0.1893628836,
0.0786091909,
0.5083714724,
0.2056815624,
0.4620290697,
-0.1761787236,
-0.5006488562,
-0.0645547807,
-0.0106144454,
0.0782232359,
0.3012277782,
0.0582543835,
-0.2225741148,
-0.4180657268,
-0.3451646864,
-0.1373445541,
-0.212616846,
-0.0264406428,
-0.2075887471,
0.3192277253,
0.1626679897,
0.0413440391,
0.0573911704,
-0.0178871658,
0.2042736411,
0.0971606299,
0.0731194764,
-0.0063092862,
-0.3482368886,
-0.2147998661,
-0.6190567017,
0.3982363939,
0.059107285,
0.2502111495,
-0.1125881076,
0.0264825504,
0.0450298116,
-0.0094933612,
0.3792361915,
-0.0885428563,
0.0296728611,
0.2719984651,
0.0443006679,
-0.435282737,
0.05192912,
-0.2449259311,
0.1143583059,
-0.0659604967,
0.2073740959,
-0.1998901218,
-0.0538148656,
0.1953684986,
0.2936964929,
0.1380235106,
-0.0931454897,
-0.4292461276,
-0.4469645023,
-0.4218346179,
-0.0641520172,
0.097307615,
0.2505412996,
0.2945303023,
0.2912118137,
0.2619181573,
-0.0290739741,
0.1567690074,
-0.0027870492,
0.0268259421,
0.2493478805,
0.015803732,
0.1950422078,
-0.0052001746,
-0.1470480561,
0.5904424191,
0.0382178873,
-0.3190020323,
-0.1221299246,
0.1556508243,
0.0909771994,
0.3510749638,
-0.1618374735,
0.2359800935,
0.1832564324,
-0.0882862955,
-0.1249310225,
-0.1221573576,
0.354141742,
0.2038033009,
-0.1567084044,
-0.4649760425,
0.2240639478,
-0.0867287964,
0.0144095207,
0.2086195052,
0.1458695978,
-0.2154882252,
0.5865092278,
-0.3359625638,
0.986443758,
0.1900929362,
0.1509921998,
0.5796771049,
0.2852432728,
0.2736798525,
-0.0253482759,
0.2704004645,
0.127061516,
-0.2383605689,
-0.0637589395,
-0.0524395183,
0.4439462721,
-0.162819311,
-0.4471860528,
0.1975351423,
0.1380908936,
-0.1930737197,
-0.0888216794,
0.1502462626,
-0.3072441518,
-0.3726421297,
-0.7613899112,
0.1629415303,
-0.0892552957,
0.435919106,
-0.0329364426,
-0.0648278892,
0.0107071903,
-0.435256809,
-0.3513129652,
0.1951479614,
-0.221455887,
0.0813252777,
-0.2043267339,
0.0401034988,
0.3659396768,
0.288926065,
0.1460966319,
0.2889968753,
-0.2387265712,
0.1064586937,
-0.1322718263,
-0.3824175596,
0.1719485968,
0.1419316828,
0.28491503,
-0.2990047932,
-0.1611962318,
0.2049421221,
-0.0569149069,
0.0150961848,
0.0441520289,
0.0061555565,
-0.0307208244,
-0.1124585941,
-0.5855568647,
0.0706821606,
-0.0553050302,
-0.1381873488,
0.1358394474,
0.0243080556,
-0.4325527847,
0.0252661444,
-0.1699226797,
-0.1066303924,
-0.0793187097,
0.5607016683,
0.1880020052,
-0.1698705405,
0.3974302411,
0.3162397444,
-0.2394102067,
-0.1771647334,
-0.0958883911,
-0.3306085765,
-0.409373343,
-0.0427019969,
0.3858549297,
-0.1172360778,
-0.2525815666,
0.3071601391,
-0.0619059727,
-0.0096784309,
-0.0838890523,
-0.2928610146,
-0.1446079761,
0.4782178402,
0.0091463383,
-0.1794580817,
0.1440698802,
-0.0686892122,
0.0756364688,
0.2157727927,
-0.3266897798,
0.1565907896,
-0.072354123,
0.2035769969,
0.3105823696,
0.0021455865,
0.2390639186,
0.050242655,
0.147093147,
0.1637431234,
-0.2215701491,
-0.203201443,
0.0223944094,
0.1006707922,
0.1046750993,
-0.0168413278,
0.1903945953,
-0.0843024626,
-0.209708035,
-0.146195665,
-0.0074350075,
0.3898077011,
-0.0087415716,
0.0350728072,
-0.1786104292,
0.0307349842,
0.1790191829,
0.0001559714,
-0.1717805713,
-0.1406947076,
0.1362287402,
0.0179870389,
-0.1118766665,
-0.085612677,
-0.0397434235,
0.1052896976,
0.0032103818,
-0.0333004631,
0.2414390445,
-0.1479865611,
0.1153254211,
0.2241201252,
0.2735121548,
0.2041804492,
-0.1730300337,
-0.025972588,
0.3789481819,
0.1346419603,
-0.5750603676,
-0.1294364333,
0.2236947864,
-0.1634566635,
0.1694571823,
0.1812379509,
0.2479255944,
0.0049995282,
0.2487307638,
0.0701473728,
0.4369536936,
-0.0982133746,
0.3608632684,
0.0550134555,
0.1738199294,
-0.0835210085,
0.1091652736,
0.1809805334,
0.2475744337,
0.3581098616,
-0.5064561963,
0.239981249,
0.1221566349,
-0.0312166698,
0.1307131797,
-0.2954683602,
0.1178456917,
0.5222584605,
0.0929435715,
0.0833180323,
-0.1304380447,
0.2733746171,
-0.1937642843,
-0.0702190176,
0.0682199523,
0.2420199364,
0.0126388948,
0.1243280619,
0.0829734951,
-0.1413501799,
-0.0892258584,
-0.160589233,
0.1149319932,
-0.1106437296,
0.0178373773,
-0.0927028582,
-0.138892591,
-0.1294717044,
0.0077095125,
-0.0036962188,
0.0070393053,
-0.1362716407,
0.3819722235,
0.0402797833,
0.0134989414,
0.0545710474,
0.5289000869,
0.6157764196,
0.2225268185,
0.15397802,
0.2291838974,
-0.3454714119,
-0.040724799,
-0.0221724156,
0.2495705634,
0.258128494,
0.317458719,
0.1913503408,
0.1021733135,
-0.1908326596,
-0.1247799769,
0.3226774037,
0.1148627102,
-0.2692885995,
0.1078656018,
-0.6182479858,
-0.0192067195,
-0.250620842,
0.021313142,
-0.7599900961,
0.0891002417,
0.4706357121,
-0.0887844414,
0.0327544548,
-0.2252699882,
0.0818886161,
-0.0447080582,
0.3434867263,
0.3529573381,
0.2142375261,
0.0181446113,
-0.3278154433,
-0.7539102435,
0.1326277256,
0.0954452828,
0.1085138917,
-0.1816552728,
-0.0798447207,
0.3282209933,
0.3236335218,
-0.1485589445,
0.0536658913,
-0.2219113559,
-0.3084180951,
-0.1348038614,
0.0154725676,
-0.0775044933,
0.2150856704,
-0.1514212489,
-0.3206720054,
-0.1670662612,
-0.357237339,
0.0848187506,
-0.0512858145,
-0.0297918338,
-0.3333076239,
-0.097757645,
0.0851005018,
0.205852434,
0.3144366145,
-0.2552306354,
-0.1501884162,
-0.1613141149,
-0.2632244527,
-0.0887676999,
0.3032117784,
0.0355335213,
0.5683208108,
-0.2828614414,
0.0654950216,
-0.3003048301,
0.5356613994,
0.0982845947,
0.0040895538,
-0.2274109721,
0.3459278941,
-0.1644192785,
0.1881949455,
-0.0067116567,
0.0318457,
-0.06167344,
0.1145702377,
-0.4813276529,
-0.3261030912,
0.6847341061,
-0.5025784373,
-0.2505761087,
0.12680161,
0.3253099024,
0.1369988471,
-0.3709214926,
-0.6134665608,
0.12574628,
0.1614838839,
0.1585963964,
-0.1042657942,
0.1900280863,
-0.3713201284,
-0.2188590169,
-0.0757652298,
0.2658396363,
0.2960599959,
-0.1947958767,
0.2011628151,
-0.2912433445
]
|
https://github.com/huggingface/datasets/issues/1994 | not being able to get wikipedia es language | @lhoestq I really appreciate if you could help me providiing processed datasets, I do not really have access to enough resources to run the apache-beam and need to run the codes on these datasets. Only en/de/fr currently works, but I need all the languages more or less. thanks | Hi
I am trying to run a code with wikipedia of config 20200501.es, getting:
Traceback (most recent call last):
File "run_mlm_t5.py", line 608, in <module>
main()
File "run_mlm_t5.py", line 359, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/load.py", line 612, in load_dataset
ignore_verifications=ignore_verifications,
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 527, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 1050, in _download_and_prepare
"\n\t`{}`".format(usage_example)
datasets.builder.MissingBeamOptions: Trying to generate a dataset using Apache Beam, yet no Beam Runner or PipelineOptions() has been provided in `load_dataset` or in the builder arguments. For big datasets it has to run on large-scale data processing tools like Dataflow, Spark, etc. More information about Apache Beam runners at https://beam.apache.org/documentation/runners/capability-matrix/
If you really want to run it locally because you feel like the Dataset is small enough, you can use the local beam runner called `DirectRunner` (you may run out of memory).
Example of usage:
`load_dataset('wikipedia', '20200501.es', beam_runner='DirectRunner')`
thanks @lhoestq for any suggestion/help | 48 | not being able to get wikipedia es language
Hi
I am trying to run a code with wikipedia of config 20200501.es, getting:
Traceback (most recent call last):
File "run_mlm_t5.py", line 608, in <module>
main()
File "run_mlm_t5.py", line 359, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/load.py", line 612, in load_dataset
ignore_verifications=ignore_verifications,
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 527, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 1050, in _download_and_prepare
"\n\t`{}`".format(usage_example)
datasets.builder.MissingBeamOptions: Trying to generate a dataset using Apache Beam, yet no Beam Runner or PipelineOptions() has been provided in `load_dataset` or in the builder arguments. For big datasets it has to run on large-scale data processing tools like Dataflow, Spark, etc. More information about Apache Beam runners at https://beam.apache.org/documentation/runners/capability-matrix/
If you really want to run it locally because you feel like the Dataset is small enough, you can use the local beam runner called `DirectRunner` (you may run out of memory).
Example of usage:
`load_dataset('wikipedia', '20200501.es', beam_runner='DirectRunner')`
thanks @lhoestq for any suggestion/help
@lhoestq I really appreciate if you could help me providiing processed datasets, I do not really have access to enough resources to run the apache-beam and need to run the codes on these datasets. Only en/de/fr currently works, but I need all the languages more or less. thanks | [
-0.3421071172,
0.0471929237,
-0.1062647477,
0.0620171316,
0.2004550546,
0.1777444631,
0.1768745631,
0.3401966393,
0.1373331696,
0.0921181589,
0.4105236232,
0.3346935809,
0.0160008296,
0.3076458573,
0.1113205701,
-0.337086916,
0.0556501672,
0.0752221942,
-0.1947190017,
-0.2001762092,
-0.1320844293,
0.1037430167,
-0.2725182474,
-0.070115529,
-0.1488353163,
0.1070381775,
0.1227781028,
-0.0196700413,
-0.1926017553,
-0.1862009913,
0.0680221766,
-0.0362134688,
0.2280334234,
0.1079431772,
-0.0001084146,
0.0806313977,
0.57174474,
-0.1583803743,
-0.4590220153,
-0.1303218603,
-0.0813638419,
-0.3462580144,
0.2564503253,
-0.3715746999,
-0.3347841501,
-0.0256398991,
0.3187550306,
-0.585716188,
0.2412880659,
0.14796184,
0.2182684541,
-0.0742109567,
0.3515350521,
-0.0048843767,
0.4105974138,
0.2186064869,
-0.0360496305,
-0.1243074462,
0.0106628658,
0.0249650497,
0.0606176145,
0.4618475437,
0.047285568,
-0.1142472029,
0.3039731085,
-0.3094905317,
0.2656845748,
-0.3731162548,
0.4229691029,
0.2541316152,
1.0161632299,
-0.119701907,
0.0995205566,
0.1050668061,
0.0040742573,
0.1287817955,
0.2428363264,
0.2005353868,
-0.3642730415,
-0.079679206,
0.1134931296,
-0.2830437124,
-0.335678786,
0.3455578387,
-0.1017288864,
0.5368952751,
0.1090376675,
0.1416432709,
-0.1509758532,
-0.185288474,
0.0773014799,
-0.070780091,
0.1030773222,
0.3541414738,
-0.0930450857,
0.1351346076,
0.2457795441,
0.1751324534,
0.0443398058,
-0.0947960988,
-0.279764384,
0.191700846,
0.3685204387,
0.0461871848,
0.0220657662,
0.0323536359,
0.3556630313,
-0.1849306375,
0.205039084,
-0.0305733252,
0.0079396451,
0.0666478723,
-0.0586210378,
-0.3365758359,
-0.6453829408,
0.2325167209,
-0.0009588738,
-0.1367515177,
0.1923418343,
0.1587267816,
-0.3540837169,
-0.2998668253,
-0.005748773,
0.2017253786,
0.1522040218,
0.2051188648,
0.2833249271,
-0.0867262706,
-0.3417101204,
-0.3844317198,
-0.046640411,
0.2829634845,
-0.4593487978,
0.078052409,
0.2093623281,
0.1067859381,
0.3750687242,
-0.0355154015,
-0.0439994782,
0.0425271429,
0.1307715178,
0.0788350254,
-0.1385990083,
0.159476012,
0.1490190029,
0.2470006347,
0.1999043524,
-0.2224675119,
-0.0522183515,
0.0016837198,
-0.0903742611,
-0.1905190051,
-0.1856554747,
0.1717607826,
0.122780256,
0.1018859744,
0.0458260253,
0.3413793445,
0.1714000404,
-0.2258879542,
0.1436648965,
-0.0075096581,
-0.1345750988,
-0.0918875337,
0.3624589443,
0.372572571,
-0.6563615799,
0.1040468812,
-0.0495636538,
0.1877676398,
0.1436999589,
-0.2795009613,
-0.2813878953,
0.4237989485,
-0.0935202166,
-0.1007727012,
0.3089181185,
-0.2708931565,
0.0815910101,
0.1968428344,
0.0673346817,
-0.2822614312,
0.2371013761,
-0.1123532876,
0.0422962159,
0.1624549329,
0.1141088828,
0.1663446426,
0.1164605319,
-0.0702251419,
-0.2196754515,
-0.1222945526,
-0.0431548283,
0.1172709763,
0.2665443718,
-0.1195797548,
0.1249020547,
0.5266056061,
0.3261623681,
-0.2632549703,
0.0764249414,
0.5432158113,
-0.3122918904,
0.0806592926,
0.1918925345,
-0.0554748699,
-0.0499001816,
0.1809134632,
-0.239194721,
0.4039112926,
0.0790574774,
-0.0053861486,
-0.2668074369,
-0.05302817,
-0.2360289097,
-0.4283643961,
0.2114924341,
0.046656061,
-0.0355188549,
0.2530861497,
0.052934926,
-0.090034768,
-0.2782234848,
-0.1389368922,
-0.8122122884,
0.2934662998,
-0.2860761583,
-0.0800811127,
-0.0875597671,
0.0202555042,
0.0639514253,
-0.1236340776,
-0.2867789865,
0.1009156108,
0.1873277426,
0.0372008197,
-0.0119494889,
0.1644939631,
0.12202999,
-0.2648279667,
0.3166706264,
0.3054232895,
0.1199404523,
0.0179686714,
-0.2019149363,
0.1108081341,
0.047732722,
0.3355243802,
0.0727866217,
0.2685894668,
0.1851827502,
0.1680479348,
-0.1562814564,
-0.1591640562,
0.3021561205,
0.4226676524,
0.181898281,
-0.1457033753,
0.0370392688,
0.0772159994,
0.4515741467,
0.1048432738,
-0.0922241807,
-0.1263715178,
-0.3054102957,
-0.0594788827,
0.4833451509,
-0.1791242361,
-0.0160226338,
0.2791059911,
-0.0161975473,
0.0771190077,
-0.1511851549,
-0.1175533831,
0.4446227849,
0.0723264888,
0.5178589821,
-0.025720587,
-0.1244163439,
-0.186818853,
-0.1908413172,
-0.2879426181,
-0.1234146729,
0.284741044,
-0.3124688864,
0.2768904269,
-0.4178830981,
-0.5642530322,
-0.2084295452,
0.4299706221,
-0.3480247557,
-0.3430694342,
-0.068008244,
0.0778863654,
-0.0229111258,
0.2500192523,
0.1072105244,
-0.0355423652,
0.2320451289,
0.0074740355,
-0.221677348,
-0.5956383348,
-0.4356403649,
0.0326633081,
0.2823260128,
0.0373753235,
0.1272295713,
-0.1569059193,
-0.0672415346,
-0.1452951729,
-0.4021958113,
0.3414440751,
-0.0869846046,
-0.0084635494,
0.1166531965,
0.4272187352,
-0.1959810108,
-0.0950585306,
0.3098011315,
0.1152016222,
-0.1476500183,
-0.0692660436,
-0.084672302,
-0.0623620301,
-0.0308235977,
-0.4383018911,
-0.1505850106,
-0.3273384273,
-0.1820001751,
0.1689834148,
0.1332358718,
0.3523069918,
0.2645772398,
0.2091481984,
0.3283596337,
0.0954611152,
-0.0965573341,
-0.1593371481,
0.2170185596,
-0.3185725212,
-0.3167464137,
0.1040421575,
-0.1413132399,
0.2305063754,
0.1135404631,
-0.1463303268,
0.068031691,
0.1159728244,
0.0200545825,
-0.10132052,
0.3257168531,
0.7469017506,
-0.0126238512,
-0.0016664611,
-0.0988115966,
0.0478255115,
0.0307381041,
-0.4368046522,
0.3473179638,
0.1777924001,
0.5277689695,
-0.070148021,
0.7515988946,
0.1148355007,
0.1166794449,
0.1321655512,
-0.0752610713,
0.0661863834,
-0.272472769,
-0.1884594262,
0.292780906,
0.0403269939,
-0.3030903637,
0.3898435831,
-0.1277018189,
-0.259891212,
-0.4387769997,
0.0750476867,
0.0015999969,
-0.2447388619,
-0.0376060195,
-0.375004977,
0.3839120865,
-0.0953027084,
0.399214685,
-0.0802457705,
-0.073142074,
0.0592005067,
0.2196860611,
-0.098245874,
0.1342238933,
-0.1730975062,
-0.2497494519,
-0.3993260264,
0.2461434305,
0.0012103891,
0.1436172575,
-0.2927531302,
0.0079170614,
0.2831737399,
0.0087450566,
0.5200991631,
-0.6470439434,
-0.0973823071,
0.3353391588,
0.1921804845,
-0.6571788192,
-0.1413873285,
-0.1876772195,
0.2771171927,
0.3285014331,
-0.0478471704,
-0.3322197497,
-0.1513581276,
0.2680895925,
0.0485687964,
-0.0990991369,
0.0515201502,
-0.2417584509,
-0.1433178782,
-0.3353154063,
-0.0657824501,
-0.0996943116,
0.1351074874,
0.1002396494,
0.2149960101,
0.0813032836,
0.0262184944,
-0.1448779553,
0.2050136477,
0.1226052344,
0.1383645535,
-0.2560669482,
0.1037121937,
0.4806474745,
-0.0265889727,
-0.1080245897,
0.1851126999,
-0.2079535574,
0.1726378053,
-0.2150923014,
0.1455086172,
0.1604102403,
-0.1206460595,
-0.2393639535,
0.036479231,
-0.2542997599,
-0.0464655384,
0.0528429002,
0.2087157369,
0.081001766,
-0.1274004281,
-0.4575787485,
0.5609187484,
0.1885293722,
-0.0729711279,
0.2990117073,
-0.0156652927,
-0.4306366444,
0.4052891731,
0.4329437912,
0.8635659814,
0.0299161691,
0.0139991231,
0.1714200377,
0.1671355665,
0.6254858375,
-0.5466775298,
0.2091643512,
-0.2812896073,
0.3198874891,
-0.0952550322,
0.0653772205,
0.3957331181,
0.1431851834,
-0.2221700996,
0.3536063433,
-0.0173850395,
0.0741585344,
0.0565966405,
0.3767171204,
0.1265330762,
-0.3078525364,
-0.1175291091,
0.1213966832,
-0.1823623627,
0.282468915,
-0.2498216778,
-0.0125389742,
-0.0145074334,
-0.2449238896,
-0.4106998742,
0.0911931768,
-0.2241830677,
0.4608557224,
-0.3423032165,
-0.4593053162,
0.2506963015,
0.2268088758,
0.2354612201,
0.3696658313,
-0.373550117,
0.2001676857,
0.0150043415,
-0.2361063957,
-0.0761885643,
0.0410973206,
0.3866564333,
-0.1922807992,
-0.392267853,
0.2118116468,
-0.1738399714,
-0.0978840888,
-0.2912859023,
-0.3177188337,
0.3188325465,
0.2502018809,
0.1240342706,
0.1725910157,
-0.0860564038,
-0.1338624209,
0.1198045388,
-0.2841424048,
0.0101037975,
0.0086888354,
0.0882756934,
-0.086818248,
0.0309087541,
0.2571510971,
0.187617898,
0.0172614269,
0.5952088237,
0.3785048425,
-0.2194746733,
-0.2659062147,
0.1786084622,
-0.2292431891,
-0.0606896169,
-0.2634163499,
0.1508059204,
0.0923175588,
-0.0571265519,
0.1029205695,
-0.0157441851,
-0.1409099549,
-0.0071758903,
-0.2680374682,
-0.0209585056,
0.0223001372,
-0.163809523,
-0.0015579847,
0.1883047223,
0.1332461685,
0.2853649259,
-0.2360227108,
-0.27911672,
-0.2388354242,
-0.0223193876,
0.0453208499,
0.413713336,
-0.0166400373,
-0.2360474467,
-0.038738545,
0.1452317834,
-0.230105862,
-0.245272398,
-0.1725503802,
-0.0468345471,
0.1290072948,
-0.0397848785,
-0.1874576509,
0.1358542144,
-0.3403597474,
-0.2925050855,
-0.2041294426,
-0.1316378266,
-0.1293067485,
-0.0242856704,
0.2222882509,
-0.1042087898,
0.3449907899,
-0.2878001034,
0.0879831091,
0.034875676,
0.2938313484,
0.0511716008,
0.2495484054,
0.4168607891,
-0.1764677018,
-0.5602070689,
0.1830452234,
-0.2211460471,
0.1743837148,
0.1633222252,
-0.0828021169,
-0.0480113775,
0.0299653579,
-0.0214734152,
0.026401462,
-0.0819887817,
-0.0302334055,
0.1355874985,
0.2045514137,
-0.2827225626,
0.1239571124,
0.4220982194,
-0.0330974758,
0.0961894989,
0.0262788963,
0.2615512311,
-0.0534214526,
0.1746624857,
0.0410569645,
0.0168640073,
-0.0434479043,
0.2639891207,
0.0946072638,
0.0811475441,
0.1160610095,
-0.1200714558,
0.046419926,
0.1310728341,
0.3746131361,
0.1071260795,
0.0660358295,
0.1008158401,
0.216569066,
-0.2253759801,
-0.1409866363,
0.3062991798,
-0.1027754843,
-0.0209083464,
0.0228770897,
-0.0645729229,
0.0748564154,
0.1480170339,
-0.0637498349,
-0.3180227578,
0.1386725008,
-0.0777270272,
-0.1830139905,
-0.6274287701,
-0.2322814912,
0.0546509698,
-0.0176587123,
0.0890894458,
-0.0836973041,
-0.1092470661,
0.2026976645,
-0.035025645,
-0.2306362838,
0.6797720194,
-0.0616486035,
0.0446974784,
-0.0551759414,
0.2520871758,
-0.213234365,
-0.0712455809,
-0.170553118,
-0.0018399562,
0.1578253806,
0.2109287828,
-0.4701038897,
-0.1439928114,
-0.0009009016,
0.0189440697,
-0.0591774844,
0.0006443821,
0.0630014166,
-0.0377971567,
0.2940510213,
0.1096708924,
-0.0898017213,
-0.1984425634,
0.2169163823,
-0.007181902,
-0.0200382136,
-0.24845469,
0.0045181345,
0.1042117402,
-0.1066087335,
0.1146248579,
-0.5152722597,
0.0413267612,
0.2594000697,
0.3884595931,
-0.1085284799,
-0.2229961902,
0.0836436301,
-0.3275438845,
0.5394181609,
0.084173657,
0.1905832291,
-0.1180475205,
-0.293014884,
-0.2950971127,
0.0054534362,
-0.1695943773,
-0.3680243194,
-0.1203949675,
0.3059184849,
0.3928039968,
0.2445940077,
0.1168807447,
-0.2535528839,
-0.129804939,
0.2650592625,
-0.1438631117,
-0.1589302421,
-0.0732823685,
0.0492415056,
-0.2251884341,
-0.1773683727,
0.0832060128,
-0.019431252,
0.0752257332,
-0.2276209742,
-0.1473571807,
0.3186531961,
-0.4347778857,
0.2595772445,
0.0258949678,
0.5472241044,
0.0291318949,
-0.0422727242,
-0.1655564606,
0.2228381783,
-0.0450003445,
0.4914211631,
0.0616208538,
0.228508994,
-0.215500325,
-0.3188501894,
-0.3452576101,
0.631701231,
0.0320963934,
-0.0550998375,
-0.2490800619,
-0.0586703122,
-0.2294992805,
0.2923284173,
-0.1192452237,
0.0662925616,
-0.036652118,
0.1867339313,
-0.3581700027,
-0.2367311567,
0.338419199,
-0.4211327136,
-0.3356799483,
-0.1897146255,
0.0682090595,
-0.1494336426,
0.2929887474,
-0.1057340801,
-0.0153544126,
0.2634702325,
-0.1352270991,
-0.0167238638,
0.00014365,
0.0598573945,
0.1573979855,
-0.2458987832,
-0.4044718742,
-0.1943297684,
-0.0603254735,
-0.3799313903,
-0.2492930144
]
|
https://github.com/huggingface/datasets/issues/1994 | not being able to get wikipedia es language | Hi @dorost1234, I think I can help you a little. I’ve processed some Wikipedia datasets (Spanish inclusive) using the HF/datasets library during recent research.
@lhoestq Could you help me to upload these preprocessed datasets to Huggingface's repositories? To be more precise, I've built datasets from the following languages using the 20201201 dumps: Spanish, Portuguese, Russian, French, Japanese, Chinese, and Turkish. Process these datasets have high costs that most of the community can't afford. I think these preprocessed datasets I have could be helpful for someone without access to high-resource machines to process Wikipedia's dumps like @dorost1234
| Hi
I am trying to run a code with wikipedia of config 20200501.es, getting:
Traceback (most recent call last):
File "run_mlm_t5.py", line 608, in <module>
main()
File "run_mlm_t5.py", line 359, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/load.py", line 612, in load_dataset
ignore_verifications=ignore_verifications,
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 527, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 1050, in _download_and_prepare
"\n\t`{}`".format(usage_example)
datasets.builder.MissingBeamOptions: Trying to generate a dataset using Apache Beam, yet no Beam Runner or PipelineOptions() has been provided in `load_dataset` or in the builder arguments. For big datasets it has to run on large-scale data processing tools like Dataflow, Spark, etc. More information about Apache Beam runners at https://beam.apache.org/documentation/runners/capability-matrix/
If you really want to run it locally because you feel like the Dataset is small enough, you can use the local beam runner called `DirectRunner` (you may run out of memory).
Example of usage:
`load_dataset('wikipedia', '20200501.es', beam_runner='DirectRunner')`
thanks @lhoestq for any suggestion/help | 96 | not being able to get wikipedia es language
Hi
I am trying to run a code with wikipedia of config 20200501.es, getting:
Traceback (most recent call last):
File "run_mlm_t5.py", line 608, in <module>
main()
File "run_mlm_t5.py", line 359, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/load.py", line 612, in load_dataset
ignore_verifications=ignore_verifications,
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 527, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 1050, in _download_and_prepare
"\n\t`{}`".format(usage_example)
datasets.builder.MissingBeamOptions: Trying to generate a dataset using Apache Beam, yet no Beam Runner or PipelineOptions() has been provided in `load_dataset` or in the builder arguments. For big datasets it has to run on large-scale data processing tools like Dataflow, Spark, etc. More information about Apache Beam runners at https://beam.apache.org/documentation/runners/capability-matrix/
If you really want to run it locally because you feel like the Dataset is small enough, you can use the local beam runner called `DirectRunner` (you may run out of memory).
Example of usage:
`load_dataset('wikipedia', '20200501.es', beam_runner='DirectRunner')`
thanks @lhoestq for any suggestion/help
Hi @dorost1234, I think I can help you a little. I’ve processed some Wikipedia datasets (Spanish inclusive) using the HF/datasets library during recent research.
@lhoestq Could you help me to upload these preprocessed datasets to Huggingface's repositories? To be more precise, I've built datasets from the following languages using the 20201201 dumps: Spanish, Portuguese, Russian, French, Japanese, Chinese, and Turkish. Process these datasets have high costs that most of the community can't afford. I think these preprocessed datasets I have could be helpful for someone without access to high-resource machines to process Wikipedia's dumps like @dorost1234
| [
-0.3421071172,
0.0471929237,
-0.1062647477,
0.0620171316,
0.2004550546,
0.1777444631,
0.1768745631,
0.3401966393,
0.1373331696,
0.0921181589,
0.4105236232,
0.3346935809,
0.0160008296,
0.3076458573,
0.1113205701,
-0.337086916,
0.0556501672,
0.0752221942,
-0.1947190017,
-0.2001762092,
-0.1320844293,
0.1037430167,
-0.2725182474,
-0.070115529,
-0.1488353163,
0.1070381775,
0.1227781028,
-0.0196700413,
-0.1926017553,
-0.1862009913,
0.0680221766,
-0.0362134688,
0.2280334234,
0.1079431772,
-0.0001084146,
0.0806313977,
0.57174474,
-0.1583803743,
-0.4590220153,
-0.1303218603,
-0.0813638419,
-0.3462580144,
0.2564503253,
-0.3715746999,
-0.3347841501,
-0.0256398991,
0.3187550306,
-0.585716188,
0.2412880659,
0.14796184,
0.2182684541,
-0.0742109567,
0.3515350521,
-0.0048843767,
0.4105974138,
0.2186064869,
-0.0360496305,
-0.1243074462,
0.0106628658,
0.0249650497,
0.0606176145,
0.4618475437,
0.047285568,
-0.1142472029,
0.3039731085,
-0.3094905317,
0.2656845748,
-0.3731162548,
0.4229691029,
0.2541316152,
1.0161632299,
-0.119701907,
0.0995205566,
0.1050668061,
0.0040742573,
0.1287817955,
0.2428363264,
0.2005353868,
-0.3642730415,
-0.079679206,
0.1134931296,
-0.2830437124,
-0.335678786,
0.3455578387,
-0.1017288864,
0.5368952751,
0.1090376675,
0.1416432709,
-0.1509758532,
-0.185288474,
0.0773014799,
-0.070780091,
0.1030773222,
0.3541414738,
-0.0930450857,
0.1351346076,
0.2457795441,
0.1751324534,
0.0443398058,
-0.0947960988,
-0.279764384,
0.191700846,
0.3685204387,
0.0461871848,
0.0220657662,
0.0323536359,
0.3556630313,
-0.1849306375,
0.205039084,
-0.0305733252,
0.0079396451,
0.0666478723,
-0.0586210378,
-0.3365758359,
-0.6453829408,
0.2325167209,
-0.0009588738,
-0.1367515177,
0.1923418343,
0.1587267816,
-0.3540837169,
-0.2998668253,
-0.005748773,
0.2017253786,
0.1522040218,
0.2051188648,
0.2833249271,
-0.0867262706,
-0.3417101204,
-0.3844317198,
-0.046640411,
0.2829634845,
-0.4593487978,
0.078052409,
0.2093623281,
0.1067859381,
0.3750687242,
-0.0355154015,
-0.0439994782,
0.0425271429,
0.1307715178,
0.0788350254,
-0.1385990083,
0.159476012,
0.1490190029,
0.2470006347,
0.1999043524,
-0.2224675119,
-0.0522183515,
0.0016837198,
-0.0903742611,
-0.1905190051,
-0.1856554747,
0.1717607826,
0.122780256,
0.1018859744,
0.0458260253,
0.3413793445,
0.1714000404,
-0.2258879542,
0.1436648965,
-0.0075096581,
-0.1345750988,
-0.0918875337,
0.3624589443,
0.372572571,
-0.6563615799,
0.1040468812,
-0.0495636538,
0.1877676398,
0.1436999589,
-0.2795009613,
-0.2813878953,
0.4237989485,
-0.0935202166,
-0.1007727012,
0.3089181185,
-0.2708931565,
0.0815910101,
0.1968428344,
0.0673346817,
-0.2822614312,
0.2371013761,
-0.1123532876,
0.0422962159,
0.1624549329,
0.1141088828,
0.1663446426,
0.1164605319,
-0.0702251419,
-0.2196754515,
-0.1222945526,
-0.0431548283,
0.1172709763,
0.2665443718,
-0.1195797548,
0.1249020547,
0.5266056061,
0.3261623681,
-0.2632549703,
0.0764249414,
0.5432158113,
-0.3122918904,
0.0806592926,
0.1918925345,
-0.0554748699,
-0.0499001816,
0.1809134632,
-0.239194721,
0.4039112926,
0.0790574774,
-0.0053861486,
-0.2668074369,
-0.05302817,
-0.2360289097,
-0.4283643961,
0.2114924341,
0.046656061,
-0.0355188549,
0.2530861497,
0.052934926,
-0.090034768,
-0.2782234848,
-0.1389368922,
-0.8122122884,
0.2934662998,
-0.2860761583,
-0.0800811127,
-0.0875597671,
0.0202555042,
0.0639514253,
-0.1236340776,
-0.2867789865,
0.1009156108,
0.1873277426,
0.0372008197,
-0.0119494889,
0.1644939631,
0.12202999,
-0.2648279667,
0.3166706264,
0.3054232895,
0.1199404523,
0.0179686714,
-0.2019149363,
0.1108081341,
0.047732722,
0.3355243802,
0.0727866217,
0.2685894668,
0.1851827502,
0.1680479348,
-0.1562814564,
-0.1591640562,
0.3021561205,
0.4226676524,
0.181898281,
-0.1457033753,
0.0370392688,
0.0772159994,
0.4515741467,
0.1048432738,
-0.0922241807,
-0.1263715178,
-0.3054102957,
-0.0594788827,
0.4833451509,
-0.1791242361,
-0.0160226338,
0.2791059911,
-0.0161975473,
0.0771190077,
-0.1511851549,
-0.1175533831,
0.4446227849,
0.0723264888,
0.5178589821,
-0.025720587,
-0.1244163439,
-0.186818853,
-0.1908413172,
-0.2879426181,
-0.1234146729,
0.284741044,
-0.3124688864,
0.2768904269,
-0.4178830981,
-0.5642530322,
-0.2084295452,
0.4299706221,
-0.3480247557,
-0.3430694342,
-0.068008244,
0.0778863654,
-0.0229111258,
0.2500192523,
0.1072105244,
-0.0355423652,
0.2320451289,
0.0074740355,
-0.221677348,
-0.5956383348,
-0.4356403649,
0.0326633081,
0.2823260128,
0.0373753235,
0.1272295713,
-0.1569059193,
-0.0672415346,
-0.1452951729,
-0.4021958113,
0.3414440751,
-0.0869846046,
-0.0084635494,
0.1166531965,
0.4272187352,
-0.1959810108,
-0.0950585306,
0.3098011315,
0.1152016222,
-0.1476500183,
-0.0692660436,
-0.084672302,
-0.0623620301,
-0.0308235977,
-0.4383018911,
-0.1505850106,
-0.3273384273,
-0.1820001751,
0.1689834148,
0.1332358718,
0.3523069918,
0.2645772398,
0.2091481984,
0.3283596337,
0.0954611152,
-0.0965573341,
-0.1593371481,
0.2170185596,
-0.3185725212,
-0.3167464137,
0.1040421575,
-0.1413132399,
0.2305063754,
0.1135404631,
-0.1463303268,
0.068031691,
0.1159728244,
0.0200545825,
-0.10132052,
0.3257168531,
0.7469017506,
-0.0126238512,
-0.0016664611,
-0.0988115966,
0.0478255115,
0.0307381041,
-0.4368046522,
0.3473179638,
0.1777924001,
0.5277689695,
-0.070148021,
0.7515988946,
0.1148355007,
0.1166794449,
0.1321655512,
-0.0752610713,
0.0661863834,
-0.272472769,
-0.1884594262,
0.292780906,
0.0403269939,
-0.3030903637,
0.3898435831,
-0.1277018189,
-0.259891212,
-0.4387769997,
0.0750476867,
0.0015999969,
-0.2447388619,
-0.0376060195,
-0.375004977,
0.3839120865,
-0.0953027084,
0.399214685,
-0.0802457705,
-0.073142074,
0.0592005067,
0.2196860611,
-0.098245874,
0.1342238933,
-0.1730975062,
-0.2497494519,
-0.3993260264,
0.2461434305,
0.0012103891,
0.1436172575,
-0.2927531302,
0.0079170614,
0.2831737399,
0.0087450566,
0.5200991631,
-0.6470439434,
-0.0973823071,
0.3353391588,
0.1921804845,
-0.6571788192,
-0.1413873285,
-0.1876772195,
0.2771171927,
0.3285014331,
-0.0478471704,
-0.3322197497,
-0.1513581276,
0.2680895925,
0.0485687964,
-0.0990991369,
0.0515201502,
-0.2417584509,
-0.1433178782,
-0.3353154063,
-0.0657824501,
-0.0996943116,
0.1351074874,
0.1002396494,
0.2149960101,
0.0813032836,
0.0262184944,
-0.1448779553,
0.2050136477,
0.1226052344,
0.1383645535,
-0.2560669482,
0.1037121937,
0.4806474745,
-0.0265889727,
-0.1080245897,
0.1851126999,
-0.2079535574,
0.1726378053,
-0.2150923014,
0.1455086172,
0.1604102403,
-0.1206460595,
-0.2393639535,
0.036479231,
-0.2542997599,
-0.0464655384,
0.0528429002,
0.2087157369,
0.081001766,
-0.1274004281,
-0.4575787485,
0.5609187484,
0.1885293722,
-0.0729711279,
0.2990117073,
-0.0156652927,
-0.4306366444,
0.4052891731,
0.4329437912,
0.8635659814,
0.0299161691,
0.0139991231,
0.1714200377,
0.1671355665,
0.6254858375,
-0.5466775298,
0.2091643512,
-0.2812896073,
0.3198874891,
-0.0952550322,
0.0653772205,
0.3957331181,
0.1431851834,
-0.2221700996,
0.3536063433,
-0.0173850395,
0.0741585344,
0.0565966405,
0.3767171204,
0.1265330762,
-0.3078525364,
-0.1175291091,
0.1213966832,
-0.1823623627,
0.282468915,
-0.2498216778,
-0.0125389742,
-0.0145074334,
-0.2449238896,
-0.4106998742,
0.0911931768,
-0.2241830677,
0.4608557224,
-0.3423032165,
-0.4593053162,
0.2506963015,
0.2268088758,
0.2354612201,
0.3696658313,
-0.373550117,
0.2001676857,
0.0150043415,
-0.2361063957,
-0.0761885643,
0.0410973206,
0.3866564333,
-0.1922807992,
-0.392267853,
0.2118116468,
-0.1738399714,
-0.0978840888,
-0.2912859023,
-0.3177188337,
0.3188325465,
0.2502018809,
0.1240342706,
0.1725910157,
-0.0860564038,
-0.1338624209,
0.1198045388,
-0.2841424048,
0.0101037975,
0.0086888354,
0.0882756934,
-0.086818248,
0.0309087541,
0.2571510971,
0.187617898,
0.0172614269,
0.5952088237,
0.3785048425,
-0.2194746733,
-0.2659062147,
0.1786084622,
-0.2292431891,
-0.0606896169,
-0.2634163499,
0.1508059204,
0.0923175588,
-0.0571265519,
0.1029205695,
-0.0157441851,
-0.1409099549,
-0.0071758903,
-0.2680374682,
-0.0209585056,
0.0223001372,
-0.163809523,
-0.0015579847,
0.1883047223,
0.1332461685,
0.2853649259,
-0.2360227108,
-0.27911672,
-0.2388354242,
-0.0223193876,
0.0453208499,
0.413713336,
-0.0166400373,
-0.2360474467,
-0.038738545,
0.1452317834,
-0.230105862,
-0.245272398,
-0.1725503802,
-0.0468345471,
0.1290072948,
-0.0397848785,
-0.1874576509,
0.1358542144,
-0.3403597474,
-0.2925050855,
-0.2041294426,
-0.1316378266,
-0.1293067485,
-0.0242856704,
0.2222882509,
-0.1042087898,
0.3449907899,
-0.2878001034,
0.0879831091,
0.034875676,
0.2938313484,
0.0511716008,
0.2495484054,
0.4168607891,
-0.1764677018,
-0.5602070689,
0.1830452234,
-0.2211460471,
0.1743837148,
0.1633222252,
-0.0828021169,
-0.0480113775,
0.0299653579,
-0.0214734152,
0.026401462,
-0.0819887817,
-0.0302334055,
0.1355874985,
0.2045514137,
-0.2827225626,
0.1239571124,
0.4220982194,
-0.0330974758,
0.0961894989,
0.0262788963,
0.2615512311,
-0.0534214526,
0.1746624857,
0.0410569645,
0.0168640073,
-0.0434479043,
0.2639891207,
0.0946072638,
0.0811475441,
0.1160610095,
-0.1200714558,
0.046419926,
0.1310728341,
0.3746131361,
0.1071260795,
0.0660358295,
0.1008158401,
0.216569066,
-0.2253759801,
-0.1409866363,
0.3062991798,
-0.1027754843,
-0.0209083464,
0.0228770897,
-0.0645729229,
0.0748564154,
0.1480170339,
-0.0637498349,
-0.3180227578,
0.1386725008,
-0.0777270272,
-0.1830139905,
-0.6274287701,
-0.2322814912,
0.0546509698,
-0.0176587123,
0.0890894458,
-0.0836973041,
-0.1092470661,
0.2026976645,
-0.035025645,
-0.2306362838,
0.6797720194,
-0.0616486035,
0.0446974784,
-0.0551759414,
0.2520871758,
-0.213234365,
-0.0712455809,
-0.170553118,
-0.0018399562,
0.1578253806,
0.2109287828,
-0.4701038897,
-0.1439928114,
-0.0009009016,
0.0189440697,
-0.0591774844,
0.0006443821,
0.0630014166,
-0.0377971567,
0.2940510213,
0.1096708924,
-0.0898017213,
-0.1984425634,
0.2169163823,
-0.007181902,
-0.0200382136,
-0.24845469,
0.0045181345,
0.1042117402,
-0.1066087335,
0.1146248579,
-0.5152722597,
0.0413267612,
0.2594000697,
0.3884595931,
-0.1085284799,
-0.2229961902,
0.0836436301,
-0.3275438845,
0.5394181609,
0.084173657,
0.1905832291,
-0.1180475205,
-0.293014884,
-0.2950971127,
0.0054534362,
-0.1695943773,
-0.3680243194,
-0.1203949675,
0.3059184849,
0.3928039968,
0.2445940077,
0.1168807447,
-0.2535528839,
-0.129804939,
0.2650592625,
-0.1438631117,
-0.1589302421,
-0.0732823685,
0.0492415056,
-0.2251884341,
-0.1773683727,
0.0832060128,
-0.019431252,
0.0752257332,
-0.2276209742,
-0.1473571807,
0.3186531961,
-0.4347778857,
0.2595772445,
0.0258949678,
0.5472241044,
0.0291318949,
-0.0422727242,
-0.1655564606,
0.2228381783,
-0.0450003445,
0.4914211631,
0.0616208538,
0.228508994,
-0.215500325,
-0.3188501894,
-0.3452576101,
0.631701231,
0.0320963934,
-0.0550998375,
-0.2490800619,
-0.0586703122,
-0.2294992805,
0.2923284173,
-0.1192452237,
0.0662925616,
-0.036652118,
0.1867339313,
-0.3581700027,
-0.2367311567,
0.338419199,
-0.4211327136,
-0.3356799483,
-0.1897146255,
0.0682090595,
-0.1494336426,
0.2929887474,
-0.1057340801,
-0.0153544126,
0.2634702325,
-0.1352270991,
-0.0167238638,
0.00014365,
0.0598573945,
0.1573979855,
-0.2458987832,
-0.4044718742,
-0.1943297684,
-0.0603254735,
-0.3799313903,
-0.2492930144
]
|
https://github.com/huggingface/datasets/issues/1994 | not being able to get wikipedia es language | Thank you so much @jonatasgrosman , I greatly appreciate your help with them.
Yes, I unfortunately does not have access to a good resource and need it for my
research. I greatly appreciate @lhoestq your help with uploading the processed datasets in huggingface datasets. This would be really helpful for some users like me with not access to high-memory GPU resources.
thank you both so much again.
On Sat, Mar 6, 2021 at 12:55 AM Jonatas Grosman <[email protected]>
wrote:
> Hi @dorost1234 <https://github.com/dorost1234>, I think I can help you a
> little. I’ve processed some Wikipedia datasets (Spanish inclusive) using
> the HF/datasets library during recent research.
>
> @lhoestq <https://github.com/lhoestq> Could you help me to upload these
> preprocessed datasets to Huggingface's repositories? To be more precise,
> I've built datasets from the following languages using the 20201201 dumps:
> Spanish, Portuguese, Russian, French, Japanese, Chinese, and Turkish.
> Process these datasets have high costs that most of the community can't
> afford. I think these preprocessed datasets I have could be helpful for
> someone without access to high-resource machines to process Wikipedia's
> dumps like @dorost1234 <https://github.com/dorost1234>
>
> —
> You are receiving this because you were mentioned.
> Reply to this email directly, view it on GitHub
> <https://github.com/huggingface/datasets/issues/1994#issuecomment-791798195>,
> or unsubscribe
> <https://github.com/notifications/unsubscribe-auth/AS37NMWMK5GFJFU3ACCJFUDTCFVNZANCNFSM4YUZIF4A>
> .
>
| Hi
I am trying to run a code with wikipedia of config 20200501.es, getting:
Traceback (most recent call last):
File "run_mlm_t5.py", line 608, in <module>
main()
File "run_mlm_t5.py", line 359, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/load.py", line 612, in load_dataset
ignore_verifications=ignore_verifications,
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 527, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 1050, in _download_and_prepare
"\n\t`{}`".format(usage_example)
datasets.builder.MissingBeamOptions: Trying to generate a dataset using Apache Beam, yet no Beam Runner or PipelineOptions() has been provided in `load_dataset` or in the builder arguments. For big datasets it has to run on large-scale data processing tools like Dataflow, Spark, etc. More information about Apache Beam runners at https://beam.apache.org/documentation/runners/capability-matrix/
If you really want to run it locally because you feel like the Dataset is small enough, you can use the local beam runner called `DirectRunner` (you may run out of memory).
Example of usage:
`load_dataset('wikipedia', '20200501.es', beam_runner='DirectRunner')`
thanks @lhoestq for any suggestion/help | 222 | not being able to get wikipedia es language
Hi
I am trying to run a code with wikipedia of config 20200501.es, getting:
Traceback (most recent call last):
File "run_mlm_t5.py", line 608, in <module>
main()
File "run_mlm_t5.py", line 359, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/load.py", line 612, in load_dataset
ignore_verifications=ignore_verifications,
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 527, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 1050, in _download_and_prepare
"\n\t`{}`".format(usage_example)
datasets.builder.MissingBeamOptions: Trying to generate a dataset using Apache Beam, yet no Beam Runner or PipelineOptions() has been provided in `load_dataset` or in the builder arguments. For big datasets it has to run on large-scale data processing tools like Dataflow, Spark, etc. More information about Apache Beam runners at https://beam.apache.org/documentation/runners/capability-matrix/
If you really want to run it locally because you feel like the Dataset is small enough, you can use the local beam runner called `DirectRunner` (you may run out of memory).
Example of usage:
`load_dataset('wikipedia', '20200501.es', beam_runner='DirectRunner')`
thanks @lhoestq for any suggestion/help
Thank you so much @jonatasgrosman , I greatly appreciate your help with them.
Yes, I unfortunately does not have access to a good resource and need it for my
research. I greatly appreciate @lhoestq your help with uploading the processed datasets in huggingface datasets. This would be really helpful for some users like me with not access to high-memory GPU resources.
thank you both so much again.
On Sat, Mar 6, 2021 at 12:55 AM Jonatas Grosman <[email protected]>
wrote:
> Hi @dorost1234 <https://github.com/dorost1234>, I think I can help you a
> little. I’ve processed some Wikipedia datasets (Spanish inclusive) using
> the HF/datasets library during recent research.
>
> @lhoestq <https://github.com/lhoestq> Could you help me to upload these
> preprocessed datasets to Huggingface's repositories? To be more precise,
> I've built datasets from the following languages using the 20201201 dumps:
> Spanish, Portuguese, Russian, French, Japanese, Chinese, and Turkish.
> Process these datasets have high costs that most of the community can't
> afford. I think these preprocessed datasets I have could be helpful for
> someone without access to high-resource machines to process Wikipedia's
> dumps like @dorost1234 <https://github.com/dorost1234>
>
> —
> You are receiving this because you were mentioned.
> Reply to this email directly, view it on GitHub
> <https://github.com/huggingface/datasets/issues/1994#issuecomment-791798195>,
> or unsubscribe
> <https://github.com/notifications/unsubscribe-auth/AS37NMWMK5GFJFU3ACCJFUDTCFVNZANCNFSM4YUZIF4A>
> .
>
| [
-0.3421071172,
0.0471929237,
-0.1062647477,
0.0620171316,
0.2004550546,
0.1777444631,
0.1768745631,
0.3401966393,
0.1373331696,
0.0921181589,
0.4105236232,
0.3346935809,
0.0160008296,
0.3076458573,
0.1113205701,
-0.337086916,
0.0556501672,
0.0752221942,
-0.1947190017,
-0.2001762092,
-0.1320844293,
0.1037430167,
-0.2725182474,
-0.070115529,
-0.1488353163,
0.1070381775,
0.1227781028,
-0.0196700413,
-0.1926017553,
-0.1862009913,
0.0680221766,
-0.0362134688,
0.2280334234,
0.1079431772,
-0.0001084146,
0.0806313977,
0.57174474,
-0.1583803743,
-0.4590220153,
-0.1303218603,
-0.0813638419,
-0.3462580144,
0.2564503253,
-0.3715746999,
-0.3347841501,
-0.0256398991,
0.3187550306,
-0.585716188,
0.2412880659,
0.14796184,
0.2182684541,
-0.0742109567,
0.3515350521,
-0.0048843767,
0.4105974138,
0.2186064869,
-0.0360496305,
-0.1243074462,
0.0106628658,
0.0249650497,
0.0606176145,
0.4618475437,
0.047285568,
-0.1142472029,
0.3039731085,
-0.3094905317,
0.2656845748,
-0.3731162548,
0.4229691029,
0.2541316152,
1.0161632299,
-0.119701907,
0.0995205566,
0.1050668061,
0.0040742573,
0.1287817955,
0.2428363264,
0.2005353868,
-0.3642730415,
-0.079679206,
0.1134931296,
-0.2830437124,
-0.335678786,
0.3455578387,
-0.1017288864,
0.5368952751,
0.1090376675,
0.1416432709,
-0.1509758532,
-0.185288474,
0.0773014799,
-0.070780091,
0.1030773222,
0.3541414738,
-0.0930450857,
0.1351346076,
0.2457795441,
0.1751324534,
0.0443398058,
-0.0947960988,
-0.279764384,
0.191700846,
0.3685204387,
0.0461871848,
0.0220657662,
0.0323536359,
0.3556630313,
-0.1849306375,
0.205039084,
-0.0305733252,
0.0079396451,
0.0666478723,
-0.0586210378,
-0.3365758359,
-0.6453829408,
0.2325167209,
-0.0009588738,
-0.1367515177,
0.1923418343,
0.1587267816,
-0.3540837169,
-0.2998668253,
-0.005748773,
0.2017253786,
0.1522040218,
0.2051188648,
0.2833249271,
-0.0867262706,
-0.3417101204,
-0.3844317198,
-0.046640411,
0.2829634845,
-0.4593487978,
0.078052409,
0.2093623281,
0.1067859381,
0.3750687242,
-0.0355154015,
-0.0439994782,
0.0425271429,
0.1307715178,
0.0788350254,
-0.1385990083,
0.159476012,
0.1490190029,
0.2470006347,
0.1999043524,
-0.2224675119,
-0.0522183515,
0.0016837198,
-0.0903742611,
-0.1905190051,
-0.1856554747,
0.1717607826,
0.122780256,
0.1018859744,
0.0458260253,
0.3413793445,
0.1714000404,
-0.2258879542,
0.1436648965,
-0.0075096581,
-0.1345750988,
-0.0918875337,
0.3624589443,
0.372572571,
-0.6563615799,
0.1040468812,
-0.0495636538,
0.1877676398,
0.1436999589,
-0.2795009613,
-0.2813878953,
0.4237989485,
-0.0935202166,
-0.1007727012,
0.3089181185,
-0.2708931565,
0.0815910101,
0.1968428344,
0.0673346817,
-0.2822614312,
0.2371013761,
-0.1123532876,
0.0422962159,
0.1624549329,
0.1141088828,
0.1663446426,
0.1164605319,
-0.0702251419,
-0.2196754515,
-0.1222945526,
-0.0431548283,
0.1172709763,
0.2665443718,
-0.1195797548,
0.1249020547,
0.5266056061,
0.3261623681,
-0.2632549703,
0.0764249414,
0.5432158113,
-0.3122918904,
0.0806592926,
0.1918925345,
-0.0554748699,
-0.0499001816,
0.1809134632,
-0.239194721,
0.4039112926,
0.0790574774,
-0.0053861486,
-0.2668074369,
-0.05302817,
-0.2360289097,
-0.4283643961,
0.2114924341,
0.046656061,
-0.0355188549,
0.2530861497,
0.052934926,
-0.090034768,
-0.2782234848,
-0.1389368922,
-0.8122122884,
0.2934662998,
-0.2860761583,
-0.0800811127,
-0.0875597671,
0.0202555042,
0.0639514253,
-0.1236340776,
-0.2867789865,
0.1009156108,
0.1873277426,
0.0372008197,
-0.0119494889,
0.1644939631,
0.12202999,
-0.2648279667,
0.3166706264,
0.3054232895,
0.1199404523,
0.0179686714,
-0.2019149363,
0.1108081341,
0.047732722,
0.3355243802,
0.0727866217,
0.2685894668,
0.1851827502,
0.1680479348,
-0.1562814564,
-0.1591640562,
0.3021561205,
0.4226676524,
0.181898281,
-0.1457033753,
0.0370392688,
0.0772159994,
0.4515741467,
0.1048432738,
-0.0922241807,
-0.1263715178,
-0.3054102957,
-0.0594788827,
0.4833451509,
-0.1791242361,
-0.0160226338,
0.2791059911,
-0.0161975473,
0.0771190077,
-0.1511851549,
-0.1175533831,
0.4446227849,
0.0723264888,
0.5178589821,
-0.025720587,
-0.1244163439,
-0.186818853,
-0.1908413172,
-0.2879426181,
-0.1234146729,
0.284741044,
-0.3124688864,
0.2768904269,
-0.4178830981,
-0.5642530322,
-0.2084295452,
0.4299706221,
-0.3480247557,
-0.3430694342,
-0.068008244,
0.0778863654,
-0.0229111258,
0.2500192523,
0.1072105244,
-0.0355423652,
0.2320451289,
0.0074740355,
-0.221677348,
-0.5956383348,
-0.4356403649,
0.0326633081,
0.2823260128,
0.0373753235,
0.1272295713,
-0.1569059193,
-0.0672415346,
-0.1452951729,
-0.4021958113,
0.3414440751,
-0.0869846046,
-0.0084635494,
0.1166531965,
0.4272187352,
-0.1959810108,
-0.0950585306,
0.3098011315,
0.1152016222,
-0.1476500183,
-0.0692660436,
-0.084672302,
-0.0623620301,
-0.0308235977,
-0.4383018911,
-0.1505850106,
-0.3273384273,
-0.1820001751,
0.1689834148,
0.1332358718,
0.3523069918,
0.2645772398,
0.2091481984,
0.3283596337,
0.0954611152,
-0.0965573341,
-0.1593371481,
0.2170185596,
-0.3185725212,
-0.3167464137,
0.1040421575,
-0.1413132399,
0.2305063754,
0.1135404631,
-0.1463303268,
0.068031691,
0.1159728244,
0.0200545825,
-0.10132052,
0.3257168531,
0.7469017506,
-0.0126238512,
-0.0016664611,
-0.0988115966,
0.0478255115,
0.0307381041,
-0.4368046522,
0.3473179638,
0.1777924001,
0.5277689695,
-0.070148021,
0.7515988946,
0.1148355007,
0.1166794449,
0.1321655512,
-0.0752610713,
0.0661863834,
-0.272472769,
-0.1884594262,
0.292780906,
0.0403269939,
-0.3030903637,
0.3898435831,
-0.1277018189,
-0.259891212,
-0.4387769997,
0.0750476867,
0.0015999969,
-0.2447388619,
-0.0376060195,
-0.375004977,
0.3839120865,
-0.0953027084,
0.399214685,
-0.0802457705,
-0.073142074,
0.0592005067,
0.2196860611,
-0.098245874,
0.1342238933,
-0.1730975062,
-0.2497494519,
-0.3993260264,
0.2461434305,
0.0012103891,
0.1436172575,
-0.2927531302,
0.0079170614,
0.2831737399,
0.0087450566,
0.5200991631,
-0.6470439434,
-0.0973823071,
0.3353391588,
0.1921804845,
-0.6571788192,
-0.1413873285,
-0.1876772195,
0.2771171927,
0.3285014331,
-0.0478471704,
-0.3322197497,
-0.1513581276,
0.2680895925,
0.0485687964,
-0.0990991369,
0.0515201502,
-0.2417584509,
-0.1433178782,
-0.3353154063,
-0.0657824501,
-0.0996943116,
0.1351074874,
0.1002396494,
0.2149960101,
0.0813032836,
0.0262184944,
-0.1448779553,
0.2050136477,
0.1226052344,
0.1383645535,
-0.2560669482,
0.1037121937,
0.4806474745,
-0.0265889727,
-0.1080245897,
0.1851126999,
-0.2079535574,
0.1726378053,
-0.2150923014,
0.1455086172,
0.1604102403,
-0.1206460595,
-0.2393639535,
0.036479231,
-0.2542997599,
-0.0464655384,
0.0528429002,
0.2087157369,
0.081001766,
-0.1274004281,
-0.4575787485,
0.5609187484,
0.1885293722,
-0.0729711279,
0.2990117073,
-0.0156652927,
-0.4306366444,
0.4052891731,
0.4329437912,
0.8635659814,
0.0299161691,
0.0139991231,
0.1714200377,
0.1671355665,
0.6254858375,
-0.5466775298,
0.2091643512,
-0.2812896073,
0.3198874891,
-0.0952550322,
0.0653772205,
0.3957331181,
0.1431851834,
-0.2221700996,
0.3536063433,
-0.0173850395,
0.0741585344,
0.0565966405,
0.3767171204,
0.1265330762,
-0.3078525364,
-0.1175291091,
0.1213966832,
-0.1823623627,
0.282468915,
-0.2498216778,
-0.0125389742,
-0.0145074334,
-0.2449238896,
-0.4106998742,
0.0911931768,
-0.2241830677,
0.4608557224,
-0.3423032165,
-0.4593053162,
0.2506963015,
0.2268088758,
0.2354612201,
0.3696658313,
-0.373550117,
0.2001676857,
0.0150043415,
-0.2361063957,
-0.0761885643,
0.0410973206,
0.3866564333,
-0.1922807992,
-0.392267853,
0.2118116468,
-0.1738399714,
-0.0978840888,
-0.2912859023,
-0.3177188337,
0.3188325465,
0.2502018809,
0.1240342706,
0.1725910157,
-0.0860564038,
-0.1338624209,
0.1198045388,
-0.2841424048,
0.0101037975,
0.0086888354,
0.0882756934,
-0.086818248,
0.0309087541,
0.2571510971,
0.187617898,
0.0172614269,
0.5952088237,
0.3785048425,
-0.2194746733,
-0.2659062147,
0.1786084622,
-0.2292431891,
-0.0606896169,
-0.2634163499,
0.1508059204,
0.0923175588,
-0.0571265519,
0.1029205695,
-0.0157441851,
-0.1409099549,
-0.0071758903,
-0.2680374682,
-0.0209585056,
0.0223001372,
-0.163809523,
-0.0015579847,
0.1883047223,
0.1332461685,
0.2853649259,
-0.2360227108,
-0.27911672,
-0.2388354242,
-0.0223193876,
0.0453208499,
0.413713336,
-0.0166400373,
-0.2360474467,
-0.038738545,
0.1452317834,
-0.230105862,
-0.245272398,
-0.1725503802,
-0.0468345471,
0.1290072948,
-0.0397848785,
-0.1874576509,
0.1358542144,
-0.3403597474,
-0.2925050855,
-0.2041294426,
-0.1316378266,
-0.1293067485,
-0.0242856704,
0.2222882509,
-0.1042087898,
0.3449907899,
-0.2878001034,
0.0879831091,
0.034875676,
0.2938313484,
0.0511716008,
0.2495484054,
0.4168607891,
-0.1764677018,
-0.5602070689,
0.1830452234,
-0.2211460471,
0.1743837148,
0.1633222252,
-0.0828021169,
-0.0480113775,
0.0299653579,
-0.0214734152,
0.026401462,
-0.0819887817,
-0.0302334055,
0.1355874985,
0.2045514137,
-0.2827225626,
0.1239571124,
0.4220982194,
-0.0330974758,
0.0961894989,
0.0262788963,
0.2615512311,
-0.0534214526,
0.1746624857,
0.0410569645,
0.0168640073,
-0.0434479043,
0.2639891207,
0.0946072638,
0.0811475441,
0.1160610095,
-0.1200714558,
0.046419926,
0.1310728341,
0.3746131361,
0.1071260795,
0.0660358295,
0.1008158401,
0.216569066,
-0.2253759801,
-0.1409866363,
0.3062991798,
-0.1027754843,
-0.0209083464,
0.0228770897,
-0.0645729229,
0.0748564154,
0.1480170339,
-0.0637498349,
-0.3180227578,
0.1386725008,
-0.0777270272,
-0.1830139905,
-0.6274287701,
-0.2322814912,
0.0546509698,
-0.0176587123,
0.0890894458,
-0.0836973041,
-0.1092470661,
0.2026976645,
-0.035025645,
-0.2306362838,
0.6797720194,
-0.0616486035,
0.0446974784,
-0.0551759414,
0.2520871758,
-0.213234365,
-0.0712455809,
-0.170553118,
-0.0018399562,
0.1578253806,
0.2109287828,
-0.4701038897,
-0.1439928114,
-0.0009009016,
0.0189440697,
-0.0591774844,
0.0006443821,
0.0630014166,
-0.0377971567,
0.2940510213,
0.1096708924,
-0.0898017213,
-0.1984425634,
0.2169163823,
-0.007181902,
-0.0200382136,
-0.24845469,
0.0045181345,
0.1042117402,
-0.1066087335,
0.1146248579,
-0.5152722597,
0.0413267612,
0.2594000697,
0.3884595931,
-0.1085284799,
-0.2229961902,
0.0836436301,
-0.3275438845,
0.5394181609,
0.084173657,
0.1905832291,
-0.1180475205,
-0.293014884,
-0.2950971127,
0.0054534362,
-0.1695943773,
-0.3680243194,
-0.1203949675,
0.3059184849,
0.3928039968,
0.2445940077,
0.1168807447,
-0.2535528839,
-0.129804939,
0.2650592625,
-0.1438631117,
-0.1589302421,
-0.0732823685,
0.0492415056,
-0.2251884341,
-0.1773683727,
0.0832060128,
-0.019431252,
0.0752257332,
-0.2276209742,
-0.1473571807,
0.3186531961,
-0.4347778857,
0.2595772445,
0.0258949678,
0.5472241044,
0.0291318949,
-0.0422727242,
-0.1655564606,
0.2228381783,
-0.0450003445,
0.4914211631,
0.0616208538,
0.228508994,
-0.215500325,
-0.3188501894,
-0.3452576101,
0.631701231,
0.0320963934,
-0.0550998375,
-0.2490800619,
-0.0586703122,
-0.2294992805,
0.2923284173,
-0.1192452237,
0.0662925616,
-0.036652118,
0.1867339313,
-0.3581700027,
-0.2367311567,
0.338419199,
-0.4211327136,
-0.3356799483,
-0.1897146255,
0.0682090595,
-0.1494336426,
0.2929887474,
-0.1057340801,
-0.0153544126,
0.2634702325,
-0.1352270991,
-0.0167238638,
0.00014365,
0.0598573945,
0.1573979855,
-0.2458987832,
-0.4044718742,
-0.1943297684,
-0.0603254735,
-0.3799313903,
-0.2492930144
]
|
https://github.com/huggingface/datasets/issues/1994 | not being able to get wikipedia es language | Hi @dorost1234, so sorry, but looking at my files here, I figure out that I've preprocessed files using the HF/datasets for all the languages previously listed by me (Portuguese, Russian, French, Japanese, Chinese, and Turkish) except the Spanish (on my tests I've used the [wikicorpus](https://www.cs.upc.edu/~nlp/wikicorpus/) instead).
Only with the Spanish Wikipedia's dump, I had the same `KeyError: '000nbsp'` problem already reported here https://github.com/huggingface/datasets/issues/577
So nowadays, even with access to a high resource machine, you couldn't be able to get Wikipedia's Spanish data using the HF/datasets :(
| Hi
I am trying to run a code with wikipedia of config 20200501.es, getting:
Traceback (most recent call last):
File "run_mlm_t5.py", line 608, in <module>
main()
File "run_mlm_t5.py", line 359, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/load.py", line 612, in load_dataset
ignore_verifications=ignore_verifications,
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 527, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 1050, in _download_and_prepare
"\n\t`{}`".format(usage_example)
datasets.builder.MissingBeamOptions: Trying to generate a dataset using Apache Beam, yet no Beam Runner or PipelineOptions() has been provided in `load_dataset` or in the builder arguments. For big datasets it has to run on large-scale data processing tools like Dataflow, Spark, etc. More information about Apache Beam runners at https://beam.apache.org/documentation/runners/capability-matrix/
If you really want to run it locally because you feel like the Dataset is small enough, you can use the local beam runner called `DirectRunner` (you may run out of memory).
Example of usage:
`load_dataset('wikipedia', '20200501.es', beam_runner='DirectRunner')`
thanks @lhoestq for any suggestion/help | 86 | not being able to get wikipedia es language
Hi
I am trying to run a code with wikipedia of config 20200501.es, getting:
Traceback (most recent call last):
File "run_mlm_t5.py", line 608, in <module>
main()
File "run_mlm_t5.py", line 359, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/load.py", line 612, in load_dataset
ignore_verifications=ignore_verifications,
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 527, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 1050, in _download_and_prepare
"\n\t`{}`".format(usage_example)
datasets.builder.MissingBeamOptions: Trying to generate a dataset using Apache Beam, yet no Beam Runner or PipelineOptions() has been provided in `load_dataset` or in the builder arguments. For big datasets it has to run on large-scale data processing tools like Dataflow, Spark, etc. More information about Apache Beam runners at https://beam.apache.org/documentation/runners/capability-matrix/
If you really want to run it locally because you feel like the Dataset is small enough, you can use the local beam runner called `DirectRunner` (you may run out of memory).
Example of usage:
`load_dataset('wikipedia', '20200501.es', beam_runner='DirectRunner')`
thanks @lhoestq for any suggestion/help
Hi @dorost1234, so sorry, but looking at my files here, I figure out that I've preprocessed files using the HF/datasets for all the languages previously listed by me (Portuguese, Russian, French, Japanese, Chinese, and Turkish) except the Spanish (on my tests I've used the [wikicorpus](https://www.cs.upc.edu/~nlp/wikicorpus/) instead).
Only with the Spanish Wikipedia's dump, I had the same `KeyError: '000nbsp'` problem already reported here https://github.com/huggingface/datasets/issues/577
So nowadays, even with access to a high resource machine, you couldn't be able to get Wikipedia's Spanish data using the HF/datasets :(
| [
-0.3421071172,
0.0471929237,
-0.1062647477,
0.0620171316,
0.2004550546,
0.1777444631,
0.1768745631,
0.3401966393,
0.1373331696,
0.0921181589,
0.4105236232,
0.3346935809,
0.0160008296,
0.3076458573,
0.1113205701,
-0.337086916,
0.0556501672,
0.0752221942,
-0.1947190017,
-0.2001762092,
-0.1320844293,
0.1037430167,
-0.2725182474,
-0.070115529,
-0.1488353163,
0.1070381775,
0.1227781028,
-0.0196700413,
-0.1926017553,
-0.1862009913,
0.0680221766,
-0.0362134688,
0.2280334234,
0.1079431772,
-0.0001084146,
0.0806313977,
0.57174474,
-0.1583803743,
-0.4590220153,
-0.1303218603,
-0.0813638419,
-0.3462580144,
0.2564503253,
-0.3715746999,
-0.3347841501,
-0.0256398991,
0.3187550306,
-0.585716188,
0.2412880659,
0.14796184,
0.2182684541,
-0.0742109567,
0.3515350521,
-0.0048843767,
0.4105974138,
0.2186064869,
-0.0360496305,
-0.1243074462,
0.0106628658,
0.0249650497,
0.0606176145,
0.4618475437,
0.047285568,
-0.1142472029,
0.3039731085,
-0.3094905317,
0.2656845748,
-0.3731162548,
0.4229691029,
0.2541316152,
1.0161632299,
-0.119701907,
0.0995205566,
0.1050668061,
0.0040742573,
0.1287817955,
0.2428363264,
0.2005353868,
-0.3642730415,
-0.079679206,
0.1134931296,
-0.2830437124,
-0.335678786,
0.3455578387,
-0.1017288864,
0.5368952751,
0.1090376675,
0.1416432709,
-0.1509758532,
-0.185288474,
0.0773014799,
-0.070780091,
0.1030773222,
0.3541414738,
-0.0930450857,
0.1351346076,
0.2457795441,
0.1751324534,
0.0443398058,
-0.0947960988,
-0.279764384,
0.191700846,
0.3685204387,
0.0461871848,
0.0220657662,
0.0323536359,
0.3556630313,
-0.1849306375,
0.205039084,
-0.0305733252,
0.0079396451,
0.0666478723,
-0.0586210378,
-0.3365758359,
-0.6453829408,
0.2325167209,
-0.0009588738,
-0.1367515177,
0.1923418343,
0.1587267816,
-0.3540837169,
-0.2998668253,
-0.005748773,
0.2017253786,
0.1522040218,
0.2051188648,
0.2833249271,
-0.0867262706,
-0.3417101204,
-0.3844317198,
-0.046640411,
0.2829634845,
-0.4593487978,
0.078052409,
0.2093623281,
0.1067859381,
0.3750687242,
-0.0355154015,
-0.0439994782,
0.0425271429,
0.1307715178,
0.0788350254,
-0.1385990083,
0.159476012,
0.1490190029,
0.2470006347,
0.1999043524,
-0.2224675119,
-0.0522183515,
0.0016837198,
-0.0903742611,
-0.1905190051,
-0.1856554747,
0.1717607826,
0.122780256,
0.1018859744,
0.0458260253,
0.3413793445,
0.1714000404,
-0.2258879542,
0.1436648965,
-0.0075096581,
-0.1345750988,
-0.0918875337,
0.3624589443,
0.372572571,
-0.6563615799,
0.1040468812,
-0.0495636538,
0.1877676398,
0.1436999589,
-0.2795009613,
-0.2813878953,
0.4237989485,
-0.0935202166,
-0.1007727012,
0.3089181185,
-0.2708931565,
0.0815910101,
0.1968428344,
0.0673346817,
-0.2822614312,
0.2371013761,
-0.1123532876,
0.0422962159,
0.1624549329,
0.1141088828,
0.1663446426,
0.1164605319,
-0.0702251419,
-0.2196754515,
-0.1222945526,
-0.0431548283,
0.1172709763,
0.2665443718,
-0.1195797548,
0.1249020547,
0.5266056061,
0.3261623681,
-0.2632549703,
0.0764249414,
0.5432158113,
-0.3122918904,
0.0806592926,
0.1918925345,
-0.0554748699,
-0.0499001816,
0.1809134632,
-0.239194721,
0.4039112926,
0.0790574774,
-0.0053861486,
-0.2668074369,
-0.05302817,
-0.2360289097,
-0.4283643961,
0.2114924341,
0.046656061,
-0.0355188549,
0.2530861497,
0.052934926,
-0.090034768,
-0.2782234848,
-0.1389368922,
-0.8122122884,
0.2934662998,
-0.2860761583,
-0.0800811127,
-0.0875597671,
0.0202555042,
0.0639514253,
-0.1236340776,
-0.2867789865,
0.1009156108,
0.1873277426,
0.0372008197,
-0.0119494889,
0.1644939631,
0.12202999,
-0.2648279667,
0.3166706264,
0.3054232895,
0.1199404523,
0.0179686714,
-0.2019149363,
0.1108081341,
0.047732722,
0.3355243802,
0.0727866217,
0.2685894668,
0.1851827502,
0.1680479348,
-0.1562814564,
-0.1591640562,
0.3021561205,
0.4226676524,
0.181898281,
-0.1457033753,
0.0370392688,
0.0772159994,
0.4515741467,
0.1048432738,
-0.0922241807,
-0.1263715178,
-0.3054102957,
-0.0594788827,
0.4833451509,
-0.1791242361,
-0.0160226338,
0.2791059911,
-0.0161975473,
0.0771190077,
-0.1511851549,
-0.1175533831,
0.4446227849,
0.0723264888,
0.5178589821,
-0.025720587,
-0.1244163439,
-0.186818853,
-0.1908413172,
-0.2879426181,
-0.1234146729,
0.284741044,
-0.3124688864,
0.2768904269,
-0.4178830981,
-0.5642530322,
-0.2084295452,
0.4299706221,
-0.3480247557,
-0.3430694342,
-0.068008244,
0.0778863654,
-0.0229111258,
0.2500192523,
0.1072105244,
-0.0355423652,
0.2320451289,
0.0074740355,
-0.221677348,
-0.5956383348,
-0.4356403649,
0.0326633081,
0.2823260128,
0.0373753235,
0.1272295713,
-0.1569059193,
-0.0672415346,
-0.1452951729,
-0.4021958113,
0.3414440751,
-0.0869846046,
-0.0084635494,
0.1166531965,
0.4272187352,
-0.1959810108,
-0.0950585306,
0.3098011315,
0.1152016222,
-0.1476500183,
-0.0692660436,
-0.084672302,
-0.0623620301,
-0.0308235977,
-0.4383018911,
-0.1505850106,
-0.3273384273,
-0.1820001751,
0.1689834148,
0.1332358718,
0.3523069918,
0.2645772398,
0.2091481984,
0.3283596337,
0.0954611152,
-0.0965573341,
-0.1593371481,
0.2170185596,
-0.3185725212,
-0.3167464137,
0.1040421575,
-0.1413132399,
0.2305063754,
0.1135404631,
-0.1463303268,
0.068031691,
0.1159728244,
0.0200545825,
-0.10132052,
0.3257168531,
0.7469017506,
-0.0126238512,
-0.0016664611,
-0.0988115966,
0.0478255115,
0.0307381041,
-0.4368046522,
0.3473179638,
0.1777924001,
0.5277689695,
-0.070148021,
0.7515988946,
0.1148355007,
0.1166794449,
0.1321655512,
-0.0752610713,
0.0661863834,
-0.272472769,
-0.1884594262,
0.292780906,
0.0403269939,
-0.3030903637,
0.3898435831,
-0.1277018189,
-0.259891212,
-0.4387769997,
0.0750476867,
0.0015999969,
-0.2447388619,
-0.0376060195,
-0.375004977,
0.3839120865,
-0.0953027084,
0.399214685,
-0.0802457705,
-0.073142074,
0.0592005067,
0.2196860611,
-0.098245874,
0.1342238933,
-0.1730975062,
-0.2497494519,
-0.3993260264,
0.2461434305,
0.0012103891,
0.1436172575,
-0.2927531302,
0.0079170614,
0.2831737399,
0.0087450566,
0.5200991631,
-0.6470439434,
-0.0973823071,
0.3353391588,
0.1921804845,
-0.6571788192,
-0.1413873285,
-0.1876772195,
0.2771171927,
0.3285014331,
-0.0478471704,
-0.3322197497,
-0.1513581276,
0.2680895925,
0.0485687964,
-0.0990991369,
0.0515201502,
-0.2417584509,
-0.1433178782,
-0.3353154063,
-0.0657824501,
-0.0996943116,
0.1351074874,
0.1002396494,
0.2149960101,
0.0813032836,
0.0262184944,
-0.1448779553,
0.2050136477,
0.1226052344,
0.1383645535,
-0.2560669482,
0.1037121937,
0.4806474745,
-0.0265889727,
-0.1080245897,
0.1851126999,
-0.2079535574,
0.1726378053,
-0.2150923014,
0.1455086172,
0.1604102403,
-0.1206460595,
-0.2393639535,
0.036479231,
-0.2542997599,
-0.0464655384,
0.0528429002,
0.2087157369,
0.081001766,
-0.1274004281,
-0.4575787485,
0.5609187484,
0.1885293722,
-0.0729711279,
0.2990117073,
-0.0156652927,
-0.4306366444,
0.4052891731,
0.4329437912,
0.8635659814,
0.0299161691,
0.0139991231,
0.1714200377,
0.1671355665,
0.6254858375,
-0.5466775298,
0.2091643512,
-0.2812896073,
0.3198874891,
-0.0952550322,
0.0653772205,
0.3957331181,
0.1431851834,
-0.2221700996,
0.3536063433,
-0.0173850395,
0.0741585344,
0.0565966405,
0.3767171204,
0.1265330762,
-0.3078525364,
-0.1175291091,
0.1213966832,
-0.1823623627,
0.282468915,
-0.2498216778,
-0.0125389742,
-0.0145074334,
-0.2449238896,
-0.4106998742,
0.0911931768,
-0.2241830677,
0.4608557224,
-0.3423032165,
-0.4593053162,
0.2506963015,
0.2268088758,
0.2354612201,
0.3696658313,
-0.373550117,
0.2001676857,
0.0150043415,
-0.2361063957,
-0.0761885643,
0.0410973206,
0.3866564333,
-0.1922807992,
-0.392267853,
0.2118116468,
-0.1738399714,
-0.0978840888,
-0.2912859023,
-0.3177188337,
0.3188325465,
0.2502018809,
0.1240342706,
0.1725910157,
-0.0860564038,
-0.1338624209,
0.1198045388,
-0.2841424048,
0.0101037975,
0.0086888354,
0.0882756934,
-0.086818248,
0.0309087541,
0.2571510971,
0.187617898,
0.0172614269,
0.5952088237,
0.3785048425,
-0.2194746733,
-0.2659062147,
0.1786084622,
-0.2292431891,
-0.0606896169,
-0.2634163499,
0.1508059204,
0.0923175588,
-0.0571265519,
0.1029205695,
-0.0157441851,
-0.1409099549,
-0.0071758903,
-0.2680374682,
-0.0209585056,
0.0223001372,
-0.163809523,
-0.0015579847,
0.1883047223,
0.1332461685,
0.2853649259,
-0.2360227108,
-0.27911672,
-0.2388354242,
-0.0223193876,
0.0453208499,
0.413713336,
-0.0166400373,
-0.2360474467,
-0.038738545,
0.1452317834,
-0.230105862,
-0.245272398,
-0.1725503802,
-0.0468345471,
0.1290072948,
-0.0397848785,
-0.1874576509,
0.1358542144,
-0.3403597474,
-0.2925050855,
-0.2041294426,
-0.1316378266,
-0.1293067485,
-0.0242856704,
0.2222882509,
-0.1042087898,
0.3449907899,
-0.2878001034,
0.0879831091,
0.034875676,
0.2938313484,
0.0511716008,
0.2495484054,
0.4168607891,
-0.1764677018,
-0.5602070689,
0.1830452234,
-0.2211460471,
0.1743837148,
0.1633222252,
-0.0828021169,
-0.0480113775,
0.0299653579,
-0.0214734152,
0.026401462,
-0.0819887817,
-0.0302334055,
0.1355874985,
0.2045514137,
-0.2827225626,
0.1239571124,
0.4220982194,
-0.0330974758,
0.0961894989,
0.0262788963,
0.2615512311,
-0.0534214526,
0.1746624857,
0.0410569645,
0.0168640073,
-0.0434479043,
0.2639891207,
0.0946072638,
0.0811475441,
0.1160610095,
-0.1200714558,
0.046419926,
0.1310728341,
0.3746131361,
0.1071260795,
0.0660358295,
0.1008158401,
0.216569066,
-0.2253759801,
-0.1409866363,
0.3062991798,
-0.1027754843,
-0.0209083464,
0.0228770897,
-0.0645729229,
0.0748564154,
0.1480170339,
-0.0637498349,
-0.3180227578,
0.1386725008,
-0.0777270272,
-0.1830139905,
-0.6274287701,
-0.2322814912,
0.0546509698,
-0.0176587123,
0.0890894458,
-0.0836973041,
-0.1092470661,
0.2026976645,
-0.035025645,
-0.2306362838,
0.6797720194,
-0.0616486035,
0.0446974784,
-0.0551759414,
0.2520871758,
-0.213234365,
-0.0712455809,
-0.170553118,
-0.0018399562,
0.1578253806,
0.2109287828,
-0.4701038897,
-0.1439928114,
-0.0009009016,
0.0189440697,
-0.0591774844,
0.0006443821,
0.0630014166,
-0.0377971567,
0.2940510213,
0.1096708924,
-0.0898017213,
-0.1984425634,
0.2169163823,
-0.007181902,
-0.0200382136,
-0.24845469,
0.0045181345,
0.1042117402,
-0.1066087335,
0.1146248579,
-0.5152722597,
0.0413267612,
0.2594000697,
0.3884595931,
-0.1085284799,
-0.2229961902,
0.0836436301,
-0.3275438845,
0.5394181609,
0.084173657,
0.1905832291,
-0.1180475205,
-0.293014884,
-0.2950971127,
0.0054534362,
-0.1695943773,
-0.3680243194,
-0.1203949675,
0.3059184849,
0.3928039968,
0.2445940077,
0.1168807447,
-0.2535528839,
-0.129804939,
0.2650592625,
-0.1438631117,
-0.1589302421,
-0.0732823685,
0.0492415056,
-0.2251884341,
-0.1773683727,
0.0832060128,
-0.019431252,
0.0752257332,
-0.2276209742,
-0.1473571807,
0.3186531961,
-0.4347778857,
0.2595772445,
0.0258949678,
0.5472241044,
0.0291318949,
-0.0422727242,
-0.1655564606,
0.2228381783,
-0.0450003445,
0.4914211631,
0.0616208538,
0.228508994,
-0.215500325,
-0.3188501894,
-0.3452576101,
0.631701231,
0.0320963934,
-0.0550998375,
-0.2490800619,
-0.0586703122,
-0.2294992805,
0.2923284173,
-0.1192452237,
0.0662925616,
-0.036652118,
0.1867339313,
-0.3581700027,
-0.2367311567,
0.338419199,
-0.4211327136,
-0.3356799483,
-0.1897146255,
0.0682090595,
-0.1494336426,
0.2929887474,
-0.1057340801,
-0.0153544126,
0.2634702325,
-0.1352270991,
-0.0167238638,
0.00014365,
0.0598573945,
0.1573979855,
-0.2458987832,
-0.4044718742,
-0.1943297684,
-0.0603254735,
-0.3799313903,
-0.2492930144
]
|
https://github.com/huggingface/datasets/issues/1994 | not being able to get wikipedia es language | Thanks a lot for the information and help. This would be great to have
these datasets.
@lhoestq <https://github.com/lhoestq> Do you know a way I could get
smaller amount of these data like 1 GBtype of each language to deal with
computatioanl requirements? thanks
On Sat, Mar 6, 2021 at 5:36 PM Jonatas Grosman <[email protected]>
wrote:
> Hi @dorost1234 <https://github.com/dorost1234>, so sorry, but looking at
> my files here, I figure out that I've preprocessed files using the
> HF/datasets for all the languages previously listed by me (Portuguese,
> Russian, French, Japanese, Chinese, and Turkish) except the Spanish (on my
> tests I've used the wikicorpus <https://www.cs.upc.edu/~nlp/wikicorpus/>
> instead).
>
> Only with the Spanish Wikipedia's dump, I had the same KeyError: '000nbsp'
> problem already reported here #577
> <https://github.com/huggingface/datasets/issues/577>
>
> So nowadays, even with access to a high resource machine, you couldn't be
> able to get Wikipedia's Spanish data using the HF/datasets :(
>
> —
> You are receiving this because you were mentioned.
> Reply to this email directly, view it on GitHub
> <https://github.com/huggingface/datasets/issues/1994#issuecomment-791985546>,
> or unsubscribe
> <https://github.com/notifications/unsubscribe-auth/AS37NMWMO7WOHWLOROPD6Q3TCJKXPANCNFSM4YUZIF4A>
> .
>
| Hi
I am trying to run a code with wikipedia of config 20200501.es, getting:
Traceback (most recent call last):
File "run_mlm_t5.py", line 608, in <module>
main()
File "run_mlm_t5.py", line 359, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/load.py", line 612, in load_dataset
ignore_verifications=ignore_verifications,
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 527, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 1050, in _download_and_prepare
"\n\t`{}`".format(usage_example)
datasets.builder.MissingBeamOptions: Trying to generate a dataset using Apache Beam, yet no Beam Runner or PipelineOptions() has been provided in `load_dataset` or in the builder arguments. For big datasets it has to run on large-scale data processing tools like Dataflow, Spark, etc. More information about Apache Beam runners at https://beam.apache.org/documentation/runners/capability-matrix/
If you really want to run it locally because you feel like the Dataset is small enough, you can use the local beam runner called `DirectRunner` (you may run out of memory).
Example of usage:
`load_dataset('wikipedia', '20200501.es', beam_runner='DirectRunner')`
thanks @lhoestq for any suggestion/help | 189 | not being able to get wikipedia es language
Hi
I am trying to run a code with wikipedia of config 20200501.es, getting:
Traceback (most recent call last):
File "run_mlm_t5.py", line 608, in <module>
main()
File "run_mlm_t5.py", line 359, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/load.py", line 612, in load_dataset
ignore_verifications=ignore_verifications,
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 527, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 1050, in _download_and_prepare
"\n\t`{}`".format(usage_example)
datasets.builder.MissingBeamOptions: Trying to generate a dataset using Apache Beam, yet no Beam Runner or PipelineOptions() has been provided in `load_dataset` or in the builder arguments. For big datasets it has to run on large-scale data processing tools like Dataflow, Spark, etc. More information about Apache Beam runners at https://beam.apache.org/documentation/runners/capability-matrix/
If you really want to run it locally because you feel like the Dataset is small enough, you can use the local beam runner called `DirectRunner` (you may run out of memory).
Example of usage:
`load_dataset('wikipedia', '20200501.es', beam_runner='DirectRunner')`
thanks @lhoestq for any suggestion/help
Thanks a lot for the information and help. This would be great to have
these datasets.
@lhoestq <https://github.com/lhoestq> Do you know a way I could get
smaller amount of these data like 1 GBtype of each language to deal with
computatioanl requirements? thanks
On Sat, Mar 6, 2021 at 5:36 PM Jonatas Grosman <[email protected]>
wrote:
> Hi @dorost1234 <https://github.com/dorost1234>, so sorry, but looking at
> my files here, I figure out that I've preprocessed files using the
> HF/datasets for all the languages previously listed by me (Portuguese,
> Russian, French, Japanese, Chinese, and Turkish) except the Spanish (on my
> tests I've used the wikicorpus <https://www.cs.upc.edu/~nlp/wikicorpus/>
> instead).
>
> Only with the Spanish Wikipedia's dump, I had the same KeyError: '000nbsp'
> problem already reported here #577
> <https://github.com/huggingface/datasets/issues/577>
>
> So nowadays, even with access to a high resource machine, you couldn't be
> able to get Wikipedia's Spanish data using the HF/datasets :(
>
> —
> You are receiving this because you were mentioned.
> Reply to this email directly, view it on GitHub
> <https://github.com/huggingface/datasets/issues/1994#issuecomment-791985546>,
> or unsubscribe
> <https://github.com/notifications/unsubscribe-auth/AS37NMWMO7WOHWLOROPD6Q3TCJKXPANCNFSM4YUZIF4A>
> .
>
| [
-0.3421071172,
0.0471929237,
-0.1062647477,
0.0620171316,
0.2004550546,
0.1777444631,
0.1768745631,
0.3401966393,
0.1373331696,
0.0921181589,
0.4105236232,
0.3346935809,
0.0160008296,
0.3076458573,
0.1113205701,
-0.337086916,
0.0556501672,
0.0752221942,
-0.1947190017,
-0.2001762092,
-0.1320844293,
0.1037430167,
-0.2725182474,
-0.070115529,
-0.1488353163,
0.1070381775,
0.1227781028,
-0.0196700413,
-0.1926017553,
-0.1862009913,
0.0680221766,
-0.0362134688,
0.2280334234,
0.1079431772,
-0.0001084146,
0.0806313977,
0.57174474,
-0.1583803743,
-0.4590220153,
-0.1303218603,
-0.0813638419,
-0.3462580144,
0.2564503253,
-0.3715746999,
-0.3347841501,
-0.0256398991,
0.3187550306,
-0.585716188,
0.2412880659,
0.14796184,
0.2182684541,
-0.0742109567,
0.3515350521,
-0.0048843767,
0.4105974138,
0.2186064869,
-0.0360496305,
-0.1243074462,
0.0106628658,
0.0249650497,
0.0606176145,
0.4618475437,
0.047285568,
-0.1142472029,
0.3039731085,
-0.3094905317,
0.2656845748,
-0.3731162548,
0.4229691029,
0.2541316152,
1.0161632299,
-0.119701907,
0.0995205566,
0.1050668061,
0.0040742573,
0.1287817955,
0.2428363264,
0.2005353868,
-0.3642730415,
-0.079679206,
0.1134931296,
-0.2830437124,
-0.335678786,
0.3455578387,
-0.1017288864,
0.5368952751,
0.1090376675,
0.1416432709,
-0.1509758532,
-0.185288474,
0.0773014799,
-0.070780091,
0.1030773222,
0.3541414738,
-0.0930450857,
0.1351346076,
0.2457795441,
0.1751324534,
0.0443398058,
-0.0947960988,
-0.279764384,
0.191700846,
0.3685204387,
0.0461871848,
0.0220657662,
0.0323536359,
0.3556630313,
-0.1849306375,
0.205039084,
-0.0305733252,
0.0079396451,
0.0666478723,
-0.0586210378,
-0.3365758359,
-0.6453829408,
0.2325167209,
-0.0009588738,
-0.1367515177,
0.1923418343,
0.1587267816,
-0.3540837169,
-0.2998668253,
-0.005748773,
0.2017253786,
0.1522040218,
0.2051188648,
0.2833249271,
-0.0867262706,
-0.3417101204,
-0.3844317198,
-0.046640411,
0.2829634845,
-0.4593487978,
0.078052409,
0.2093623281,
0.1067859381,
0.3750687242,
-0.0355154015,
-0.0439994782,
0.0425271429,
0.1307715178,
0.0788350254,
-0.1385990083,
0.159476012,
0.1490190029,
0.2470006347,
0.1999043524,
-0.2224675119,
-0.0522183515,
0.0016837198,
-0.0903742611,
-0.1905190051,
-0.1856554747,
0.1717607826,
0.122780256,
0.1018859744,
0.0458260253,
0.3413793445,
0.1714000404,
-0.2258879542,
0.1436648965,
-0.0075096581,
-0.1345750988,
-0.0918875337,
0.3624589443,
0.372572571,
-0.6563615799,
0.1040468812,
-0.0495636538,
0.1877676398,
0.1436999589,
-0.2795009613,
-0.2813878953,
0.4237989485,
-0.0935202166,
-0.1007727012,
0.3089181185,
-0.2708931565,
0.0815910101,
0.1968428344,
0.0673346817,
-0.2822614312,
0.2371013761,
-0.1123532876,
0.0422962159,
0.1624549329,
0.1141088828,
0.1663446426,
0.1164605319,
-0.0702251419,
-0.2196754515,
-0.1222945526,
-0.0431548283,
0.1172709763,
0.2665443718,
-0.1195797548,
0.1249020547,
0.5266056061,
0.3261623681,
-0.2632549703,
0.0764249414,
0.5432158113,
-0.3122918904,
0.0806592926,
0.1918925345,
-0.0554748699,
-0.0499001816,
0.1809134632,
-0.239194721,
0.4039112926,
0.0790574774,
-0.0053861486,
-0.2668074369,
-0.05302817,
-0.2360289097,
-0.4283643961,
0.2114924341,
0.046656061,
-0.0355188549,
0.2530861497,
0.052934926,
-0.090034768,
-0.2782234848,
-0.1389368922,
-0.8122122884,
0.2934662998,
-0.2860761583,
-0.0800811127,
-0.0875597671,
0.0202555042,
0.0639514253,
-0.1236340776,
-0.2867789865,
0.1009156108,
0.1873277426,
0.0372008197,
-0.0119494889,
0.1644939631,
0.12202999,
-0.2648279667,
0.3166706264,
0.3054232895,
0.1199404523,
0.0179686714,
-0.2019149363,
0.1108081341,
0.047732722,
0.3355243802,
0.0727866217,
0.2685894668,
0.1851827502,
0.1680479348,
-0.1562814564,
-0.1591640562,
0.3021561205,
0.4226676524,
0.181898281,
-0.1457033753,
0.0370392688,
0.0772159994,
0.4515741467,
0.1048432738,
-0.0922241807,
-0.1263715178,
-0.3054102957,
-0.0594788827,
0.4833451509,
-0.1791242361,
-0.0160226338,
0.2791059911,
-0.0161975473,
0.0771190077,
-0.1511851549,
-0.1175533831,
0.4446227849,
0.0723264888,
0.5178589821,
-0.025720587,
-0.1244163439,
-0.186818853,
-0.1908413172,
-0.2879426181,
-0.1234146729,
0.284741044,
-0.3124688864,
0.2768904269,
-0.4178830981,
-0.5642530322,
-0.2084295452,
0.4299706221,
-0.3480247557,
-0.3430694342,
-0.068008244,
0.0778863654,
-0.0229111258,
0.2500192523,
0.1072105244,
-0.0355423652,
0.2320451289,
0.0074740355,
-0.221677348,
-0.5956383348,
-0.4356403649,
0.0326633081,
0.2823260128,
0.0373753235,
0.1272295713,
-0.1569059193,
-0.0672415346,
-0.1452951729,
-0.4021958113,
0.3414440751,
-0.0869846046,
-0.0084635494,
0.1166531965,
0.4272187352,
-0.1959810108,
-0.0950585306,
0.3098011315,
0.1152016222,
-0.1476500183,
-0.0692660436,
-0.084672302,
-0.0623620301,
-0.0308235977,
-0.4383018911,
-0.1505850106,
-0.3273384273,
-0.1820001751,
0.1689834148,
0.1332358718,
0.3523069918,
0.2645772398,
0.2091481984,
0.3283596337,
0.0954611152,
-0.0965573341,
-0.1593371481,
0.2170185596,
-0.3185725212,
-0.3167464137,
0.1040421575,
-0.1413132399,
0.2305063754,
0.1135404631,
-0.1463303268,
0.068031691,
0.1159728244,
0.0200545825,
-0.10132052,
0.3257168531,
0.7469017506,
-0.0126238512,
-0.0016664611,
-0.0988115966,
0.0478255115,
0.0307381041,
-0.4368046522,
0.3473179638,
0.1777924001,
0.5277689695,
-0.070148021,
0.7515988946,
0.1148355007,
0.1166794449,
0.1321655512,
-0.0752610713,
0.0661863834,
-0.272472769,
-0.1884594262,
0.292780906,
0.0403269939,
-0.3030903637,
0.3898435831,
-0.1277018189,
-0.259891212,
-0.4387769997,
0.0750476867,
0.0015999969,
-0.2447388619,
-0.0376060195,
-0.375004977,
0.3839120865,
-0.0953027084,
0.399214685,
-0.0802457705,
-0.073142074,
0.0592005067,
0.2196860611,
-0.098245874,
0.1342238933,
-0.1730975062,
-0.2497494519,
-0.3993260264,
0.2461434305,
0.0012103891,
0.1436172575,
-0.2927531302,
0.0079170614,
0.2831737399,
0.0087450566,
0.5200991631,
-0.6470439434,
-0.0973823071,
0.3353391588,
0.1921804845,
-0.6571788192,
-0.1413873285,
-0.1876772195,
0.2771171927,
0.3285014331,
-0.0478471704,
-0.3322197497,
-0.1513581276,
0.2680895925,
0.0485687964,
-0.0990991369,
0.0515201502,
-0.2417584509,
-0.1433178782,
-0.3353154063,
-0.0657824501,
-0.0996943116,
0.1351074874,
0.1002396494,
0.2149960101,
0.0813032836,
0.0262184944,
-0.1448779553,
0.2050136477,
0.1226052344,
0.1383645535,
-0.2560669482,
0.1037121937,
0.4806474745,
-0.0265889727,
-0.1080245897,
0.1851126999,
-0.2079535574,
0.1726378053,
-0.2150923014,
0.1455086172,
0.1604102403,
-0.1206460595,
-0.2393639535,
0.036479231,
-0.2542997599,
-0.0464655384,
0.0528429002,
0.2087157369,
0.081001766,
-0.1274004281,
-0.4575787485,
0.5609187484,
0.1885293722,
-0.0729711279,
0.2990117073,
-0.0156652927,
-0.4306366444,
0.4052891731,
0.4329437912,
0.8635659814,
0.0299161691,
0.0139991231,
0.1714200377,
0.1671355665,
0.6254858375,
-0.5466775298,
0.2091643512,
-0.2812896073,
0.3198874891,
-0.0952550322,
0.0653772205,
0.3957331181,
0.1431851834,
-0.2221700996,
0.3536063433,
-0.0173850395,
0.0741585344,
0.0565966405,
0.3767171204,
0.1265330762,
-0.3078525364,
-0.1175291091,
0.1213966832,
-0.1823623627,
0.282468915,
-0.2498216778,
-0.0125389742,
-0.0145074334,
-0.2449238896,
-0.4106998742,
0.0911931768,
-0.2241830677,
0.4608557224,
-0.3423032165,
-0.4593053162,
0.2506963015,
0.2268088758,
0.2354612201,
0.3696658313,
-0.373550117,
0.2001676857,
0.0150043415,
-0.2361063957,
-0.0761885643,
0.0410973206,
0.3866564333,
-0.1922807992,
-0.392267853,
0.2118116468,
-0.1738399714,
-0.0978840888,
-0.2912859023,
-0.3177188337,
0.3188325465,
0.2502018809,
0.1240342706,
0.1725910157,
-0.0860564038,
-0.1338624209,
0.1198045388,
-0.2841424048,
0.0101037975,
0.0086888354,
0.0882756934,
-0.086818248,
0.0309087541,
0.2571510971,
0.187617898,
0.0172614269,
0.5952088237,
0.3785048425,
-0.2194746733,
-0.2659062147,
0.1786084622,
-0.2292431891,
-0.0606896169,
-0.2634163499,
0.1508059204,
0.0923175588,
-0.0571265519,
0.1029205695,
-0.0157441851,
-0.1409099549,
-0.0071758903,
-0.2680374682,
-0.0209585056,
0.0223001372,
-0.163809523,
-0.0015579847,
0.1883047223,
0.1332461685,
0.2853649259,
-0.2360227108,
-0.27911672,
-0.2388354242,
-0.0223193876,
0.0453208499,
0.413713336,
-0.0166400373,
-0.2360474467,
-0.038738545,
0.1452317834,
-0.230105862,
-0.245272398,
-0.1725503802,
-0.0468345471,
0.1290072948,
-0.0397848785,
-0.1874576509,
0.1358542144,
-0.3403597474,
-0.2925050855,
-0.2041294426,
-0.1316378266,
-0.1293067485,
-0.0242856704,
0.2222882509,
-0.1042087898,
0.3449907899,
-0.2878001034,
0.0879831091,
0.034875676,
0.2938313484,
0.0511716008,
0.2495484054,
0.4168607891,
-0.1764677018,
-0.5602070689,
0.1830452234,
-0.2211460471,
0.1743837148,
0.1633222252,
-0.0828021169,
-0.0480113775,
0.0299653579,
-0.0214734152,
0.026401462,
-0.0819887817,
-0.0302334055,
0.1355874985,
0.2045514137,
-0.2827225626,
0.1239571124,
0.4220982194,
-0.0330974758,
0.0961894989,
0.0262788963,
0.2615512311,
-0.0534214526,
0.1746624857,
0.0410569645,
0.0168640073,
-0.0434479043,
0.2639891207,
0.0946072638,
0.0811475441,
0.1160610095,
-0.1200714558,
0.046419926,
0.1310728341,
0.3746131361,
0.1071260795,
0.0660358295,
0.1008158401,
0.216569066,
-0.2253759801,
-0.1409866363,
0.3062991798,
-0.1027754843,
-0.0209083464,
0.0228770897,
-0.0645729229,
0.0748564154,
0.1480170339,
-0.0637498349,
-0.3180227578,
0.1386725008,
-0.0777270272,
-0.1830139905,
-0.6274287701,
-0.2322814912,
0.0546509698,
-0.0176587123,
0.0890894458,
-0.0836973041,
-0.1092470661,
0.2026976645,
-0.035025645,
-0.2306362838,
0.6797720194,
-0.0616486035,
0.0446974784,
-0.0551759414,
0.2520871758,
-0.213234365,
-0.0712455809,
-0.170553118,
-0.0018399562,
0.1578253806,
0.2109287828,
-0.4701038897,
-0.1439928114,
-0.0009009016,
0.0189440697,
-0.0591774844,
0.0006443821,
0.0630014166,
-0.0377971567,
0.2940510213,
0.1096708924,
-0.0898017213,
-0.1984425634,
0.2169163823,
-0.007181902,
-0.0200382136,
-0.24845469,
0.0045181345,
0.1042117402,
-0.1066087335,
0.1146248579,
-0.5152722597,
0.0413267612,
0.2594000697,
0.3884595931,
-0.1085284799,
-0.2229961902,
0.0836436301,
-0.3275438845,
0.5394181609,
0.084173657,
0.1905832291,
-0.1180475205,
-0.293014884,
-0.2950971127,
0.0054534362,
-0.1695943773,
-0.3680243194,
-0.1203949675,
0.3059184849,
0.3928039968,
0.2445940077,
0.1168807447,
-0.2535528839,
-0.129804939,
0.2650592625,
-0.1438631117,
-0.1589302421,
-0.0732823685,
0.0492415056,
-0.2251884341,
-0.1773683727,
0.0832060128,
-0.019431252,
0.0752257332,
-0.2276209742,
-0.1473571807,
0.3186531961,
-0.4347778857,
0.2595772445,
0.0258949678,
0.5472241044,
0.0291318949,
-0.0422727242,
-0.1655564606,
0.2228381783,
-0.0450003445,
0.4914211631,
0.0616208538,
0.228508994,
-0.215500325,
-0.3188501894,
-0.3452576101,
0.631701231,
0.0320963934,
-0.0550998375,
-0.2490800619,
-0.0586703122,
-0.2294992805,
0.2923284173,
-0.1192452237,
0.0662925616,
-0.036652118,
0.1867339313,
-0.3581700027,
-0.2367311567,
0.338419199,
-0.4211327136,
-0.3356799483,
-0.1897146255,
0.0682090595,
-0.1494336426,
0.2929887474,
-0.1057340801,
-0.0153544126,
0.2634702325,
-0.1352270991,
-0.0167238638,
0.00014365,
0.0598573945,
0.1573979855,
-0.2458987832,
-0.4044718742,
-0.1943297684,
-0.0603254735,
-0.3799313903,
-0.2492930144
]
|
https://github.com/huggingface/datasets/issues/1994 | not being able to get wikipedia es language | Hi ! As mentioned above the Spanish configuration have parsing issues from `mwparserfromhell`. I haven't tested with the latest `mwparserfromhell` >=0.6 though. Which version of `mwparserfromhell` are you using ?
> @lhoestq Could you help me to upload these preprocessed datasets to Huggingface's repositories? To be more precise, I've built datasets from the following languages using the 20201201 dumps: Spanish, Portuguese, Russian, French, Japanese, Chinese, and Turkish. Process these datasets have high costs that most of the community can't afford. I think these preprocessed datasets I have could be helpful for someone without access to high-resource machines to process Wikipedia's dumps like @dorost1234
That would be awesome ! Feel free to ping me on slack so we can put the processed wikipedia files on google storage with the other ones we've already preprocessed.
> Do you know a way I could get smaller amount of these data like 1 GBtype of each language to deal with computatioanl requirements? thanks
I'd suggest to copy the [wikipedia.py](https://github.com/huggingface/datasets/blob/master/datasets/wikipedia/wikipedia.py) to a new script `custom_wikipedia.py` and modify it to only download and process only a subset of the raw data files.
You can for example replace [this line](https://github.com/huggingface/datasets/blob/64e59fc45ca2134218b3e42e83fddddbe840ff74/datasets/wikipedia/wikipedia.py#L446) by:
```python
if total_bytes >= (1 << 30): # stop if the total amount of data is >= 1GB
break
else:
xml_urls.append(_base_url(lang) + fname)
```
Then you can load your custom wikipedia dataset with
```python
load_dataset("path/to/my/custom_wikipedia.py", f"{date}.{language}")
``` | Hi
I am trying to run a code with wikipedia of config 20200501.es, getting:
Traceback (most recent call last):
File "run_mlm_t5.py", line 608, in <module>
main()
File "run_mlm_t5.py", line 359, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/load.py", line 612, in load_dataset
ignore_verifications=ignore_verifications,
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 527, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 1050, in _download_and_prepare
"\n\t`{}`".format(usage_example)
datasets.builder.MissingBeamOptions: Trying to generate a dataset using Apache Beam, yet no Beam Runner or PipelineOptions() has been provided in `load_dataset` or in the builder arguments. For big datasets it has to run on large-scale data processing tools like Dataflow, Spark, etc. More information about Apache Beam runners at https://beam.apache.org/documentation/runners/capability-matrix/
If you really want to run it locally because you feel like the Dataset is small enough, you can use the local beam runner called `DirectRunner` (you may run out of memory).
Example of usage:
`load_dataset('wikipedia', '20200501.es', beam_runner='DirectRunner')`
thanks @lhoestq for any suggestion/help | 231 | not being able to get wikipedia es language
Hi
I am trying to run a code with wikipedia of config 20200501.es, getting:
Traceback (most recent call last):
File "run_mlm_t5.py", line 608, in <module>
main()
File "run_mlm_t5.py", line 359, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/load.py", line 612, in load_dataset
ignore_verifications=ignore_verifications,
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 527, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 1050, in _download_and_prepare
"\n\t`{}`".format(usage_example)
datasets.builder.MissingBeamOptions: Trying to generate a dataset using Apache Beam, yet no Beam Runner or PipelineOptions() has been provided in `load_dataset` or in the builder arguments. For big datasets it has to run on large-scale data processing tools like Dataflow, Spark, etc. More information about Apache Beam runners at https://beam.apache.org/documentation/runners/capability-matrix/
If you really want to run it locally because you feel like the Dataset is small enough, you can use the local beam runner called `DirectRunner` (you may run out of memory).
Example of usage:
`load_dataset('wikipedia', '20200501.es', beam_runner='DirectRunner')`
thanks @lhoestq for any suggestion/help
Hi ! As mentioned above the Spanish configuration have parsing issues from `mwparserfromhell`. I haven't tested with the latest `mwparserfromhell` >=0.6 though. Which version of `mwparserfromhell` are you using ?
> @lhoestq Could you help me to upload these preprocessed datasets to Huggingface's repositories? To be more precise, I've built datasets from the following languages using the 20201201 dumps: Spanish, Portuguese, Russian, French, Japanese, Chinese, and Turkish. Process these datasets have high costs that most of the community can't afford. I think these preprocessed datasets I have could be helpful for someone without access to high-resource machines to process Wikipedia's dumps like @dorost1234
That would be awesome ! Feel free to ping me on slack so we can put the processed wikipedia files on google storage with the other ones we've already preprocessed.
> Do you know a way I could get smaller amount of these data like 1 GBtype of each language to deal with computatioanl requirements? thanks
I'd suggest to copy the [wikipedia.py](https://github.com/huggingface/datasets/blob/master/datasets/wikipedia/wikipedia.py) to a new script `custom_wikipedia.py` and modify it to only download and process only a subset of the raw data files.
You can for example replace [this line](https://github.com/huggingface/datasets/blob/64e59fc45ca2134218b3e42e83fddddbe840ff74/datasets/wikipedia/wikipedia.py#L446) by:
```python
if total_bytes >= (1 << 30): # stop if the total amount of data is >= 1GB
break
else:
xml_urls.append(_base_url(lang) + fname)
```
Then you can load your custom wikipedia dataset with
```python
load_dataset("path/to/my/custom_wikipedia.py", f"{date}.{language}")
``` | [
-0.3421071172,
0.0471929237,
-0.1062647477,
0.0620171316,
0.2004550546,
0.1777444631,
0.1768745631,
0.3401966393,
0.1373331696,
0.0921181589,
0.4105236232,
0.3346935809,
0.0160008296,
0.3076458573,
0.1113205701,
-0.337086916,
0.0556501672,
0.0752221942,
-0.1947190017,
-0.2001762092,
-0.1320844293,
0.1037430167,
-0.2725182474,
-0.070115529,
-0.1488353163,
0.1070381775,
0.1227781028,
-0.0196700413,
-0.1926017553,
-0.1862009913,
0.0680221766,
-0.0362134688,
0.2280334234,
0.1079431772,
-0.0001084146,
0.0806313977,
0.57174474,
-0.1583803743,
-0.4590220153,
-0.1303218603,
-0.0813638419,
-0.3462580144,
0.2564503253,
-0.3715746999,
-0.3347841501,
-0.0256398991,
0.3187550306,
-0.585716188,
0.2412880659,
0.14796184,
0.2182684541,
-0.0742109567,
0.3515350521,
-0.0048843767,
0.4105974138,
0.2186064869,
-0.0360496305,
-0.1243074462,
0.0106628658,
0.0249650497,
0.0606176145,
0.4618475437,
0.047285568,
-0.1142472029,
0.3039731085,
-0.3094905317,
0.2656845748,
-0.3731162548,
0.4229691029,
0.2541316152,
1.0161632299,
-0.119701907,
0.0995205566,
0.1050668061,
0.0040742573,
0.1287817955,
0.2428363264,
0.2005353868,
-0.3642730415,
-0.079679206,
0.1134931296,
-0.2830437124,
-0.335678786,
0.3455578387,
-0.1017288864,
0.5368952751,
0.1090376675,
0.1416432709,
-0.1509758532,
-0.185288474,
0.0773014799,
-0.070780091,
0.1030773222,
0.3541414738,
-0.0930450857,
0.1351346076,
0.2457795441,
0.1751324534,
0.0443398058,
-0.0947960988,
-0.279764384,
0.191700846,
0.3685204387,
0.0461871848,
0.0220657662,
0.0323536359,
0.3556630313,
-0.1849306375,
0.205039084,
-0.0305733252,
0.0079396451,
0.0666478723,
-0.0586210378,
-0.3365758359,
-0.6453829408,
0.2325167209,
-0.0009588738,
-0.1367515177,
0.1923418343,
0.1587267816,
-0.3540837169,
-0.2998668253,
-0.005748773,
0.2017253786,
0.1522040218,
0.2051188648,
0.2833249271,
-0.0867262706,
-0.3417101204,
-0.3844317198,
-0.046640411,
0.2829634845,
-0.4593487978,
0.078052409,
0.2093623281,
0.1067859381,
0.3750687242,
-0.0355154015,
-0.0439994782,
0.0425271429,
0.1307715178,
0.0788350254,
-0.1385990083,
0.159476012,
0.1490190029,
0.2470006347,
0.1999043524,
-0.2224675119,
-0.0522183515,
0.0016837198,
-0.0903742611,
-0.1905190051,
-0.1856554747,
0.1717607826,
0.122780256,
0.1018859744,
0.0458260253,
0.3413793445,
0.1714000404,
-0.2258879542,
0.1436648965,
-0.0075096581,
-0.1345750988,
-0.0918875337,
0.3624589443,
0.372572571,
-0.6563615799,
0.1040468812,
-0.0495636538,
0.1877676398,
0.1436999589,
-0.2795009613,
-0.2813878953,
0.4237989485,
-0.0935202166,
-0.1007727012,
0.3089181185,
-0.2708931565,
0.0815910101,
0.1968428344,
0.0673346817,
-0.2822614312,
0.2371013761,
-0.1123532876,
0.0422962159,
0.1624549329,
0.1141088828,
0.1663446426,
0.1164605319,
-0.0702251419,
-0.2196754515,
-0.1222945526,
-0.0431548283,
0.1172709763,
0.2665443718,
-0.1195797548,
0.1249020547,
0.5266056061,
0.3261623681,
-0.2632549703,
0.0764249414,
0.5432158113,
-0.3122918904,
0.0806592926,
0.1918925345,
-0.0554748699,
-0.0499001816,
0.1809134632,
-0.239194721,
0.4039112926,
0.0790574774,
-0.0053861486,
-0.2668074369,
-0.05302817,
-0.2360289097,
-0.4283643961,
0.2114924341,
0.046656061,
-0.0355188549,
0.2530861497,
0.052934926,
-0.090034768,
-0.2782234848,
-0.1389368922,
-0.8122122884,
0.2934662998,
-0.2860761583,
-0.0800811127,
-0.0875597671,
0.0202555042,
0.0639514253,
-0.1236340776,
-0.2867789865,
0.1009156108,
0.1873277426,
0.0372008197,
-0.0119494889,
0.1644939631,
0.12202999,
-0.2648279667,
0.3166706264,
0.3054232895,
0.1199404523,
0.0179686714,
-0.2019149363,
0.1108081341,
0.047732722,
0.3355243802,
0.0727866217,
0.2685894668,
0.1851827502,
0.1680479348,
-0.1562814564,
-0.1591640562,
0.3021561205,
0.4226676524,
0.181898281,
-0.1457033753,
0.0370392688,
0.0772159994,
0.4515741467,
0.1048432738,
-0.0922241807,
-0.1263715178,
-0.3054102957,
-0.0594788827,
0.4833451509,
-0.1791242361,
-0.0160226338,
0.2791059911,
-0.0161975473,
0.0771190077,
-0.1511851549,
-0.1175533831,
0.4446227849,
0.0723264888,
0.5178589821,
-0.025720587,
-0.1244163439,
-0.186818853,
-0.1908413172,
-0.2879426181,
-0.1234146729,
0.284741044,
-0.3124688864,
0.2768904269,
-0.4178830981,
-0.5642530322,
-0.2084295452,
0.4299706221,
-0.3480247557,
-0.3430694342,
-0.068008244,
0.0778863654,
-0.0229111258,
0.2500192523,
0.1072105244,
-0.0355423652,
0.2320451289,
0.0074740355,
-0.221677348,
-0.5956383348,
-0.4356403649,
0.0326633081,
0.2823260128,
0.0373753235,
0.1272295713,
-0.1569059193,
-0.0672415346,
-0.1452951729,
-0.4021958113,
0.3414440751,
-0.0869846046,
-0.0084635494,
0.1166531965,
0.4272187352,
-0.1959810108,
-0.0950585306,
0.3098011315,
0.1152016222,
-0.1476500183,
-0.0692660436,
-0.084672302,
-0.0623620301,
-0.0308235977,
-0.4383018911,
-0.1505850106,
-0.3273384273,
-0.1820001751,
0.1689834148,
0.1332358718,
0.3523069918,
0.2645772398,
0.2091481984,
0.3283596337,
0.0954611152,
-0.0965573341,
-0.1593371481,
0.2170185596,
-0.3185725212,
-0.3167464137,
0.1040421575,
-0.1413132399,
0.2305063754,
0.1135404631,
-0.1463303268,
0.068031691,
0.1159728244,
0.0200545825,
-0.10132052,
0.3257168531,
0.7469017506,
-0.0126238512,
-0.0016664611,
-0.0988115966,
0.0478255115,
0.0307381041,
-0.4368046522,
0.3473179638,
0.1777924001,
0.5277689695,
-0.070148021,
0.7515988946,
0.1148355007,
0.1166794449,
0.1321655512,
-0.0752610713,
0.0661863834,
-0.272472769,
-0.1884594262,
0.292780906,
0.0403269939,
-0.3030903637,
0.3898435831,
-0.1277018189,
-0.259891212,
-0.4387769997,
0.0750476867,
0.0015999969,
-0.2447388619,
-0.0376060195,
-0.375004977,
0.3839120865,
-0.0953027084,
0.399214685,
-0.0802457705,
-0.073142074,
0.0592005067,
0.2196860611,
-0.098245874,
0.1342238933,
-0.1730975062,
-0.2497494519,
-0.3993260264,
0.2461434305,
0.0012103891,
0.1436172575,
-0.2927531302,
0.0079170614,
0.2831737399,
0.0087450566,
0.5200991631,
-0.6470439434,
-0.0973823071,
0.3353391588,
0.1921804845,
-0.6571788192,
-0.1413873285,
-0.1876772195,
0.2771171927,
0.3285014331,
-0.0478471704,
-0.3322197497,
-0.1513581276,
0.2680895925,
0.0485687964,
-0.0990991369,
0.0515201502,
-0.2417584509,
-0.1433178782,
-0.3353154063,
-0.0657824501,
-0.0996943116,
0.1351074874,
0.1002396494,
0.2149960101,
0.0813032836,
0.0262184944,
-0.1448779553,
0.2050136477,
0.1226052344,
0.1383645535,
-0.2560669482,
0.1037121937,
0.4806474745,
-0.0265889727,
-0.1080245897,
0.1851126999,
-0.2079535574,
0.1726378053,
-0.2150923014,
0.1455086172,
0.1604102403,
-0.1206460595,
-0.2393639535,
0.036479231,
-0.2542997599,
-0.0464655384,
0.0528429002,
0.2087157369,
0.081001766,
-0.1274004281,
-0.4575787485,
0.5609187484,
0.1885293722,
-0.0729711279,
0.2990117073,
-0.0156652927,
-0.4306366444,
0.4052891731,
0.4329437912,
0.8635659814,
0.0299161691,
0.0139991231,
0.1714200377,
0.1671355665,
0.6254858375,
-0.5466775298,
0.2091643512,
-0.2812896073,
0.3198874891,
-0.0952550322,
0.0653772205,
0.3957331181,
0.1431851834,
-0.2221700996,
0.3536063433,
-0.0173850395,
0.0741585344,
0.0565966405,
0.3767171204,
0.1265330762,
-0.3078525364,
-0.1175291091,
0.1213966832,
-0.1823623627,
0.282468915,
-0.2498216778,
-0.0125389742,
-0.0145074334,
-0.2449238896,
-0.4106998742,
0.0911931768,
-0.2241830677,
0.4608557224,
-0.3423032165,
-0.4593053162,
0.2506963015,
0.2268088758,
0.2354612201,
0.3696658313,
-0.373550117,
0.2001676857,
0.0150043415,
-0.2361063957,
-0.0761885643,
0.0410973206,
0.3866564333,
-0.1922807992,
-0.392267853,
0.2118116468,
-0.1738399714,
-0.0978840888,
-0.2912859023,
-0.3177188337,
0.3188325465,
0.2502018809,
0.1240342706,
0.1725910157,
-0.0860564038,
-0.1338624209,
0.1198045388,
-0.2841424048,
0.0101037975,
0.0086888354,
0.0882756934,
-0.086818248,
0.0309087541,
0.2571510971,
0.187617898,
0.0172614269,
0.5952088237,
0.3785048425,
-0.2194746733,
-0.2659062147,
0.1786084622,
-0.2292431891,
-0.0606896169,
-0.2634163499,
0.1508059204,
0.0923175588,
-0.0571265519,
0.1029205695,
-0.0157441851,
-0.1409099549,
-0.0071758903,
-0.2680374682,
-0.0209585056,
0.0223001372,
-0.163809523,
-0.0015579847,
0.1883047223,
0.1332461685,
0.2853649259,
-0.2360227108,
-0.27911672,
-0.2388354242,
-0.0223193876,
0.0453208499,
0.413713336,
-0.0166400373,
-0.2360474467,
-0.038738545,
0.1452317834,
-0.230105862,
-0.245272398,
-0.1725503802,
-0.0468345471,
0.1290072948,
-0.0397848785,
-0.1874576509,
0.1358542144,
-0.3403597474,
-0.2925050855,
-0.2041294426,
-0.1316378266,
-0.1293067485,
-0.0242856704,
0.2222882509,
-0.1042087898,
0.3449907899,
-0.2878001034,
0.0879831091,
0.034875676,
0.2938313484,
0.0511716008,
0.2495484054,
0.4168607891,
-0.1764677018,
-0.5602070689,
0.1830452234,
-0.2211460471,
0.1743837148,
0.1633222252,
-0.0828021169,
-0.0480113775,
0.0299653579,
-0.0214734152,
0.026401462,
-0.0819887817,
-0.0302334055,
0.1355874985,
0.2045514137,
-0.2827225626,
0.1239571124,
0.4220982194,
-0.0330974758,
0.0961894989,
0.0262788963,
0.2615512311,
-0.0534214526,
0.1746624857,
0.0410569645,
0.0168640073,
-0.0434479043,
0.2639891207,
0.0946072638,
0.0811475441,
0.1160610095,
-0.1200714558,
0.046419926,
0.1310728341,
0.3746131361,
0.1071260795,
0.0660358295,
0.1008158401,
0.216569066,
-0.2253759801,
-0.1409866363,
0.3062991798,
-0.1027754843,
-0.0209083464,
0.0228770897,
-0.0645729229,
0.0748564154,
0.1480170339,
-0.0637498349,
-0.3180227578,
0.1386725008,
-0.0777270272,
-0.1830139905,
-0.6274287701,
-0.2322814912,
0.0546509698,
-0.0176587123,
0.0890894458,
-0.0836973041,
-0.1092470661,
0.2026976645,
-0.035025645,
-0.2306362838,
0.6797720194,
-0.0616486035,
0.0446974784,
-0.0551759414,
0.2520871758,
-0.213234365,
-0.0712455809,
-0.170553118,
-0.0018399562,
0.1578253806,
0.2109287828,
-0.4701038897,
-0.1439928114,
-0.0009009016,
0.0189440697,
-0.0591774844,
0.0006443821,
0.0630014166,
-0.0377971567,
0.2940510213,
0.1096708924,
-0.0898017213,
-0.1984425634,
0.2169163823,
-0.007181902,
-0.0200382136,
-0.24845469,
0.0045181345,
0.1042117402,
-0.1066087335,
0.1146248579,
-0.5152722597,
0.0413267612,
0.2594000697,
0.3884595931,
-0.1085284799,
-0.2229961902,
0.0836436301,
-0.3275438845,
0.5394181609,
0.084173657,
0.1905832291,
-0.1180475205,
-0.293014884,
-0.2950971127,
0.0054534362,
-0.1695943773,
-0.3680243194,
-0.1203949675,
0.3059184849,
0.3928039968,
0.2445940077,
0.1168807447,
-0.2535528839,
-0.129804939,
0.2650592625,
-0.1438631117,
-0.1589302421,
-0.0732823685,
0.0492415056,
-0.2251884341,
-0.1773683727,
0.0832060128,
-0.019431252,
0.0752257332,
-0.2276209742,
-0.1473571807,
0.3186531961,
-0.4347778857,
0.2595772445,
0.0258949678,
0.5472241044,
0.0291318949,
-0.0422727242,
-0.1655564606,
0.2228381783,
-0.0450003445,
0.4914211631,
0.0616208538,
0.228508994,
-0.215500325,
-0.3188501894,
-0.3452576101,
0.631701231,
0.0320963934,
-0.0550998375,
-0.2490800619,
-0.0586703122,
-0.2294992805,
0.2923284173,
-0.1192452237,
0.0662925616,
-0.036652118,
0.1867339313,
-0.3581700027,
-0.2367311567,
0.338419199,
-0.4211327136,
-0.3356799483,
-0.1897146255,
0.0682090595,
-0.1494336426,
0.2929887474,
-0.1057340801,
-0.0153544126,
0.2634702325,
-0.1352270991,
-0.0167238638,
0.00014365,
0.0598573945,
0.1573979855,
-0.2458987832,
-0.4044718742,
-0.1943297684,
-0.0603254735,
-0.3799313903,
-0.2492930144
]
|
https://github.com/huggingface/datasets/issues/1994 | not being able to get wikipedia es language | Hi @lhoestq!
> Hi ! As mentioned above the Spanish configuration have parsing issues from mwparserfromhell. I haven't tested with the latest mwparserfromhell >=0.6 though. Which version of mwparserfromhell are you using ?
I'm using the latest mwparserfromhell version (0.6)
> That would be awesome ! Feel free to ping me on slack so we can put the processed wikipedia files on google storage with the other ones we've already preprocessed.
I'll ping you there 👍 | Hi
I am trying to run a code with wikipedia of config 20200501.es, getting:
Traceback (most recent call last):
File "run_mlm_t5.py", line 608, in <module>
main()
File "run_mlm_t5.py", line 359, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/load.py", line 612, in load_dataset
ignore_verifications=ignore_verifications,
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 527, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 1050, in _download_and_prepare
"\n\t`{}`".format(usage_example)
datasets.builder.MissingBeamOptions: Trying to generate a dataset using Apache Beam, yet no Beam Runner or PipelineOptions() has been provided in `load_dataset` or in the builder arguments. For big datasets it has to run on large-scale data processing tools like Dataflow, Spark, etc. More information about Apache Beam runners at https://beam.apache.org/documentation/runners/capability-matrix/
If you really want to run it locally because you feel like the Dataset is small enough, you can use the local beam runner called `DirectRunner` (you may run out of memory).
Example of usage:
`load_dataset('wikipedia', '20200501.es', beam_runner='DirectRunner')`
thanks @lhoestq for any suggestion/help | 76 | not being able to get wikipedia es language
Hi
I am trying to run a code with wikipedia of config 20200501.es, getting:
Traceback (most recent call last):
File "run_mlm_t5.py", line 608, in <module>
main()
File "run_mlm_t5.py", line 359, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/load.py", line 612, in load_dataset
ignore_verifications=ignore_verifications,
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 527, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 1050, in _download_and_prepare
"\n\t`{}`".format(usage_example)
datasets.builder.MissingBeamOptions: Trying to generate a dataset using Apache Beam, yet no Beam Runner or PipelineOptions() has been provided in `load_dataset` or in the builder arguments. For big datasets it has to run on large-scale data processing tools like Dataflow, Spark, etc. More information about Apache Beam runners at https://beam.apache.org/documentation/runners/capability-matrix/
If you really want to run it locally because you feel like the Dataset is small enough, you can use the local beam runner called `DirectRunner` (you may run out of memory).
Example of usage:
`load_dataset('wikipedia', '20200501.es', beam_runner='DirectRunner')`
thanks @lhoestq for any suggestion/help
Hi @lhoestq!
> Hi ! As mentioned above the Spanish configuration have parsing issues from mwparserfromhell. I haven't tested with the latest mwparserfromhell >=0.6 though. Which version of mwparserfromhell are you using ?
I'm using the latest mwparserfromhell version (0.6)
> That would be awesome ! Feel free to ping me on slack so we can put the processed wikipedia files on google storage with the other ones we've already preprocessed.
I'll ping you there 👍 | [
-0.3421071172,
0.0471929237,
-0.1062647477,
0.0620171316,
0.2004550546,
0.1777444631,
0.1768745631,
0.3401966393,
0.1373331696,
0.0921181589,
0.4105236232,
0.3346935809,
0.0160008296,
0.3076458573,
0.1113205701,
-0.337086916,
0.0556501672,
0.0752221942,
-0.1947190017,
-0.2001762092,
-0.1320844293,
0.1037430167,
-0.2725182474,
-0.070115529,
-0.1488353163,
0.1070381775,
0.1227781028,
-0.0196700413,
-0.1926017553,
-0.1862009913,
0.0680221766,
-0.0362134688,
0.2280334234,
0.1079431772,
-0.0001084146,
0.0806313977,
0.57174474,
-0.1583803743,
-0.4590220153,
-0.1303218603,
-0.0813638419,
-0.3462580144,
0.2564503253,
-0.3715746999,
-0.3347841501,
-0.0256398991,
0.3187550306,
-0.585716188,
0.2412880659,
0.14796184,
0.2182684541,
-0.0742109567,
0.3515350521,
-0.0048843767,
0.4105974138,
0.2186064869,
-0.0360496305,
-0.1243074462,
0.0106628658,
0.0249650497,
0.0606176145,
0.4618475437,
0.047285568,
-0.1142472029,
0.3039731085,
-0.3094905317,
0.2656845748,
-0.3731162548,
0.4229691029,
0.2541316152,
1.0161632299,
-0.119701907,
0.0995205566,
0.1050668061,
0.0040742573,
0.1287817955,
0.2428363264,
0.2005353868,
-0.3642730415,
-0.079679206,
0.1134931296,
-0.2830437124,
-0.335678786,
0.3455578387,
-0.1017288864,
0.5368952751,
0.1090376675,
0.1416432709,
-0.1509758532,
-0.185288474,
0.0773014799,
-0.070780091,
0.1030773222,
0.3541414738,
-0.0930450857,
0.1351346076,
0.2457795441,
0.1751324534,
0.0443398058,
-0.0947960988,
-0.279764384,
0.191700846,
0.3685204387,
0.0461871848,
0.0220657662,
0.0323536359,
0.3556630313,
-0.1849306375,
0.205039084,
-0.0305733252,
0.0079396451,
0.0666478723,
-0.0586210378,
-0.3365758359,
-0.6453829408,
0.2325167209,
-0.0009588738,
-0.1367515177,
0.1923418343,
0.1587267816,
-0.3540837169,
-0.2998668253,
-0.005748773,
0.2017253786,
0.1522040218,
0.2051188648,
0.2833249271,
-0.0867262706,
-0.3417101204,
-0.3844317198,
-0.046640411,
0.2829634845,
-0.4593487978,
0.078052409,
0.2093623281,
0.1067859381,
0.3750687242,
-0.0355154015,
-0.0439994782,
0.0425271429,
0.1307715178,
0.0788350254,
-0.1385990083,
0.159476012,
0.1490190029,
0.2470006347,
0.1999043524,
-0.2224675119,
-0.0522183515,
0.0016837198,
-0.0903742611,
-0.1905190051,
-0.1856554747,
0.1717607826,
0.122780256,
0.1018859744,
0.0458260253,
0.3413793445,
0.1714000404,
-0.2258879542,
0.1436648965,
-0.0075096581,
-0.1345750988,
-0.0918875337,
0.3624589443,
0.372572571,
-0.6563615799,
0.1040468812,
-0.0495636538,
0.1877676398,
0.1436999589,
-0.2795009613,
-0.2813878953,
0.4237989485,
-0.0935202166,
-0.1007727012,
0.3089181185,
-0.2708931565,
0.0815910101,
0.1968428344,
0.0673346817,
-0.2822614312,
0.2371013761,
-0.1123532876,
0.0422962159,
0.1624549329,
0.1141088828,
0.1663446426,
0.1164605319,
-0.0702251419,
-0.2196754515,
-0.1222945526,
-0.0431548283,
0.1172709763,
0.2665443718,
-0.1195797548,
0.1249020547,
0.5266056061,
0.3261623681,
-0.2632549703,
0.0764249414,
0.5432158113,
-0.3122918904,
0.0806592926,
0.1918925345,
-0.0554748699,
-0.0499001816,
0.1809134632,
-0.239194721,
0.4039112926,
0.0790574774,
-0.0053861486,
-0.2668074369,
-0.05302817,
-0.2360289097,
-0.4283643961,
0.2114924341,
0.046656061,
-0.0355188549,
0.2530861497,
0.052934926,
-0.090034768,
-0.2782234848,
-0.1389368922,
-0.8122122884,
0.2934662998,
-0.2860761583,
-0.0800811127,
-0.0875597671,
0.0202555042,
0.0639514253,
-0.1236340776,
-0.2867789865,
0.1009156108,
0.1873277426,
0.0372008197,
-0.0119494889,
0.1644939631,
0.12202999,
-0.2648279667,
0.3166706264,
0.3054232895,
0.1199404523,
0.0179686714,
-0.2019149363,
0.1108081341,
0.047732722,
0.3355243802,
0.0727866217,
0.2685894668,
0.1851827502,
0.1680479348,
-0.1562814564,
-0.1591640562,
0.3021561205,
0.4226676524,
0.181898281,
-0.1457033753,
0.0370392688,
0.0772159994,
0.4515741467,
0.1048432738,
-0.0922241807,
-0.1263715178,
-0.3054102957,
-0.0594788827,
0.4833451509,
-0.1791242361,
-0.0160226338,
0.2791059911,
-0.0161975473,
0.0771190077,
-0.1511851549,
-0.1175533831,
0.4446227849,
0.0723264888,
0.5178589821,
-0.025720587,
-0.1244163439,
-0.186818853,
-0.1908413172,
-0.2879426181,
-0.1234146729,
0.284741044,
-0.3124688864,
0.2768904269,
-0.4178830981,
-0.5642530322,
-0.2084295452,
0.4299706221,
-0.3480247557,
-0.3430694342,
-0.068008244,
0.0778863654,
-0.0229111258,
0.2500192523,
0.1072105244,
-0.0355423652,
0.2320451289,
0.0074740355,
-0.221677348,
-0.5956383348,
-0.4356403649,
0.0326633081,
0.2823260128,
0.0373753235,
0.1272295713,
-0.1569059193,
-0.0672415346,
-0.1452951729,
-0.4021958113,
0.3414440751,
-0.0869846046,
-0.0084635494,
0.1166531965,
0.4272187352,
-0.1959810108,
-0.0950585306,
0.3098011315,
0.1152016222,
-0.1476500183,
-0.0692660436,
-0.084672302,
-0.0623620301,
-0.0308235977,
-0.4383018911,
-0.1505850106,
-0.3273384273,
-0.1820001751,
0.1689834148,
0.1332358718,
0.3523069918,
0.2645772398,
0.2091481984,
0.3283596337,
0.0954611152,
-0.0965573341,
-0.1593371481,
0.2170185596,
-0.3185725212,
-0.3167464137,
0.1040421575,
-0.1413132399,
0.2305063754,
0.1135404631,
-0.1463303268,
0.068031691,
0.1159728244,
0.0200545825,
-0.10132052,
0.3257168531,
0.7469017506,
-0.0126238512,
-0.0016664611,
-0.0988115966,
0.0478255115,
0.0307381041,
-0.4368046522,
0.3473179638,
0.1777924001,
0.5277689695,
-0.070148021,
0.7515988946,
0.1148355007,
0.1166794449,
0.1321655512,
-0.0752610713,
0.0661863834,
-0.272472769,
-0.1884594262,
0.292780906,
0.0403269939,
-0.3030903637,
0.3898435831,
-0.1277018189,
-0.259891212,
-0.4387769997,
0.0750476867,
0.0015999969,
-0.2447388619,
-0.0376060195,
-0.375004977,
0.3839120865,
-0.0953027084,
0.399214685,
-0.0802457705,
-0.073142074,
0.0592005067,
0.2196860611,
-0.098245874,
0.1342238933,
-0.1730975062,
-0.2497494519,
-0.3993260264,
0.2461434305,
0.0012103891,
0.1436172575,
-0.2927531302,
0.0079170614,
0.2831737399,
0.0087450566,
0.5200991631,
-0.6470439434,
-0.0973823071,
0.3353391588,
0.1921804845,
-0.6571788192,
-0.1413873285,
-0.1876772195,
0.2771171927,
0.3285014331,
-0.0478471704,
-0.3322197497,
-0.1513581276,
0.2680895925,
0.0485687964,
-0.0990991369,
0.0515201502,
-0.2417584509,
-0.1433178782,
-0.3353154063,
-0.0657824501,
-0.0996943116,
0.1351074874,
0.1002396494,
0.2149960101,
0.0813032836,
0.0262184944,
-0.1448779553,
0.2050136477,
0.1226052344,
0.1383645535,
-0.2560669482,
0.1037121937,
0.4806474745,
-0.0265889727,
-0.1080245897,
0.1851126999,
-0.2079535574,
0.1726378053,
-0.2150923014,
0.1455086172,
0.1604102403,
-0.1206460595,
-0.2393639535,
0.036479231,
-0.2542997599,
-0.0464655384,
0.0528429002,
0.2087157369,
0.081001766,
-0.1274004281,
-0.4575787485,
0.5609187484,
0.1885293722,
-0.0729711279,
0.2990117073,
-0.0156652927,
-0.4306366444,
0.4052891731,
0.4329437912,
0.8635659814,
0.0299161691,
0.0139991231,
0.1714200377,
0.1671355665,
0.6254858375,
-0.5466775298,
0.2091643512,
-0.2812896073,
0.3198874891,
-0.0952550322,
0.0653772205,
0.3957331181,
0.1431851834,
-0.2221700996,
0.3536063433,
-0.0173850395,
0.0741585344,
0.0565966405,
0.3767171204,
0.1265330762,
-0.3078525364,
-0.1175291091,
0.1213966832,
-0.1823623627,
0.282468915,
-0.2498216778,
-0.0125389742,
-0.0145074334,
-0.2449238896,
-0.4106998742,
0.0911931768,
-0.2241830677,
0.4608557224,
-0.3423032165,
-0.4593053162,
0.2506963015,
0.2268088758,
0.2354612201,
0.3696658313,
-0.373550117,
0.2001676857,
0.0150043415,
-0.2361063957,
-0.0761885643,
0.0410973206,
0.3866564333,
-0.1922807992,
-0.392267853,
0.2118116468,
-0.1738399714,
-0.0978840888,
-0.2912859023,
-0.3177188337,
0.3188325465,
0.2502018809,
0.1240342706,
0.1725910157,
-0.0860564038,
-0.1338624209,
0.1198045388,
-0.2841424048,
0.0101037975,
0.0086888354,
0.0882756934,
-0.086818248,
0.0309087541,
0.2571510971,
0.187617898,
0.0172614269,
0.5952088237,
0.3785048425,
-0.2194746733,
-0.2659062147,
0.1786084622,
-0.2292431891,
-0.0606896169,
-0.2634163499,
0.1508059204,
0.0923175588,
-0.0571265519,
0.1029205695,
-0.0157441851,
-0.1409099549,
-0.0071758903,
-0.2680374682,
-0.0209585056,
0.0223001372,
-0.163809523,
-0.0015579847,
0.1883047223,
0.1332461685,
0.2853649259,
-0.2360227108,
-0.27911672,
-0.2388354242,
-0.0223193876,
0.0453208499,
0.413713336,
-0.0166400373,
-0.2360474467,
-0.038738545,
0.1452317834,
-0.230105862,
-0.245272398,
-0.1725503802,
-0.0468345471,
0.1290072948,
-0.0397848785,
-0.1874576509,
0.1358542144,
-0.3403597474,
-0.2925050855,
-0.2041294426,
-0.1316378266,
-0.1293067485,
-0.0242856704,
0.2222882509,
-0.1042087898,
0.3449907899,
-0.2878001034,
0.0879831091,
0.034875676,
0.2938313484,
0.0511716008,
0.2495484054,
0.4168607891,
-0.1764677018,
-0.5602070689,
0.1830452234,
-0.2211460471,
0.1743837148,
0.1633222252,
-0.0828021169,
-0.0480113775,
0.0299653579,
-0.0214734152,
0.026401462,
-0.0819887817,
-0.0302334055,
0.1355874985,
0.2045514137,
-0.2827225626,
0.1239571124,
0.4220982194,
-0.0330974758,
0.0961894989,
0.0262788963,
0.2615512311,
-0.0534214526,
0.1746624857,
0.0410569645,
0.0168640073,
-0.0434479043,
0.2639891207,
0.0946072638,
0.0811475441,
0.1160610095,
-0.1200714558,
0.046419926,
0.1310728341,
0.3746131361,
0.1071260795,
0.0660358295,
0.1008158401,
0.216569066,
-0.2253759801,
-0.1409866363,
0.3062991798,
-0.1027754843,
-0.0209083464,
0.0228770897,
-0.0645729229,
0.0748564154,
0.1480170339,
-0.0637498349,
-0.3180227578,
0.1386725008,
-0.0777270272,
-0.1830139905,
-0.6274287701,
-0.2322814912,
0.0546509698,
-0.0176587123,
0.0890894458,
-0.0836973041,
-0.1092470661,
0.2026976645,
-0.035025645,
-0.2306362838,
0.6797720194,
-0.0616486035,
0.0446974784,
-0.0551759414,
0.2520871758,
-0.213234365,
-0.0712455809,
-0.170553118,
-0.0018399562,
0.1578253806,
0.2109287828,
-0.4701038897,
-0.1439928114,
-0.0009009016,
0.0189440697,
-0.0591774844,
0.0006443821,
0.0630014166,
-0.0377971567,
0.2940510213,
0.1096708924,
-0.0898017213,
-0.1984425634,
0.2169163823,
-0.007181902,
-0.0200382136,
-0.24845469,
0.0045181345,
0.1042117402,
-0.1066087335,
0.1146248579,
-0.5152722597,
0.0413267612,
0.2594000697,
0.3884595931,
-0.1085284799,
-0.2229961902,
0.0836436301,
-0.3275438845,
0.5394181609,
0.084173657,
0.1905832291,
-0.1180475205,
-0.293014884,
-0.2950971127,
0.0054534362,
-0.1695943773,
-0.3680243194,
-0.1203949675,
0.3059184849,
0.3928039968,
0.2445940077,
0.1168807447,
-0.2535528839,
-0.129804939,
0.2650592625,
-0.1438631117,
-0.1589302421,
-0.0732823685,
0.0492415056,
-0.2251884341,
-0.1773683727,
0.0832060128,
-0.019431252,
0.0752257332,
-0.2276209742,
-0.1473571807,
0.3186531961,
-0.4347778857,
0.2595772445,
0.0258949678,
0.5472241044,
0.0291318949,
-0.0422727242,
-0.1655564606,
0.2228381783,
-0.0450003445,
0.4914211631,
0.0616208538,
0.228508994,
-0.215500325,
-0.3188501894,
-0.3452576101,
0.631701231,
0.0320963934,
-0.0550998375,
-0.2490800619,
-0.0586703122,
-0.2294992805,
0.2923284173,
-0.1192452237,
0.0662925616,
-0.036652118,
0.1867339313,
-0.3581700027,
-0.2367311567,
0.338419199,
-0.4211327136,
-0.3356799483,
-0.1897146255,
0.0682090595,
-0.1494336426,
0.2929887474,
-0.1057340801,
-0.0153544126,
0.2634702325,
-0.1352270991,
-0.0167238638,
0.00014365,
0.0598573945,
0.1573979855,
-0.2458987832,
-0.4044718742,
-0.1943297684,
-0.0603254735,
-0.3799313903,
-0.2492930144
]
|
https://github.com/huggingface/datasets/issues/1994 | not being able to get wikipedia es language | Thank you so much @jonatasgrosman and @lhoestq this would be a great help. I am really thankful to you both and to wonderful Huggingface dataset library allowing us to train models at scale. | Hi
I am trying to run a code with wikipedia of config 20200501.es, getting:
Traceback (most recent call last):
File "run_mlm_t5.py", line 608, in <module>
main()
File "run_mlm_t5.py", line 359, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/load.py", line 612, in load_dataset
ignore_verifications=ignore_verifications,
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 527, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 1050, in _download_and_prepare
"\n\t`{}`".format(usage_example)
datasets.builder.MissingBeamOptions: Trying to generate a dataset using Apache Beam, yet no Beam Runner or PipelineOptions() has been provided in `load_dataset` or in the builder arguments. For big datasets it has to run on large-scale data processing tools like Dataflow, Spark, etc. More information about Apache Beam runners at https://beam.apache.org/documentation/runners/capability-matrix/
If you really want to run it locally because you feel like the Dataset is small enough, you can use the local beam runner called `DirectRunner` (you may run out of memory).
Example of usage:
`load_dataset('wikipedia', '20200501.es', beam_runner='DirectRunner')`
thanks @lhoestq for any suggestion/help | 33 | not being able to get wikipedia es language
Hi
I am trying to run a code with wikipedia of config 20200501.es, getting:
Traceback (most recent call last):
File "run_mlm_t5.py", line 608, in <module>
main()
File "run_mlm_t5.py", line 359, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/load.py", line 612, in load_dataset
ignore_verifications=ignore_verifications,
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 527, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/dara/libs/anaconda3/envs/success432/lib/python3.7/site-packages/datasets-1.2.1-py3.7.egg/datasets/builder.py", line 1050, in _download_and_prepare
"\n\t`{}`".format(usage_example)
datasets.builder.MissingBeamOptions: Trying to generate a dataset using Apache Beam, yet no Beam Runner or PipelineOptions() has been provided in `load_dataset` or in the builder arguments. For big datasets it has to run on large-scale data processing tools like Dataflow, Spark, etc. More information about Apache Beam runners at https://beam.apache.org/documentation/runners/capability-matrix/
If you really want to run it locally because you feel like the Dataset is small enough, you can use the local beam runner called `DirectRunner` (you may run out of memory).
Example of usage:
`load_dataset('wikipedia', '20200501.es', beam_runner='DirectRunner')`
thanks @lhoestq for any suggestion/help
Thank you so much @jonatasgrosman and @lhoestq this would be a great help. I am really thankful to you both and to wonderful Huggingface dataset library allowing us to train models at scale. | [
-0.3421071172,
0.0471929237,
-0.1062647477,
0.0620171316,
0.2004550546,
0.1777444631,
0.1768745631,
0.3401966393,
0.1373331696,
0.0921181589,
0.4105236232,
0.3346935809,
0.0160008296,
0.3076458573,
0.1113205701,
-0.337086916,
0.0556501672,
0.0752221942,
-0.1947190017,
-0.2001762092,
-0.1320844293,
0.1037430167,
-0.2725182474,
-0.070115529,
-0.1488353163,
0.1070381775,
0.1227781028,
-0.0196700413,
-0.1926017553,
-0.1862009913,
0.0680221766,
-0.0362134688,
0.2280334234,
0.1079431772,
-0.0001084146,
0.0806313977,
0.57174474,
-0.1583803743,
-0.4590220153,
-0.1303218603,
-0.0813638419,
-0.3462580144,
0.2564503253,
-0.3715746999,
-0.3347841501,
-0.0256398991,
0.3187550306,
-0.585716188,
0.2412880659,
0.14796184,
0.2182684541,
-0.0742109567,
0.3515350521,
-0.0048843767,
0.4105974138,
0.2186064869,
-0.0360496305,
-0.1243074462,
0.0106628658,
0.0249650497,
0.0606176145,
0.4618475437,
0.047285568,
-0.1142472029,
0.3039731085,
-0.3094905317,
0.2656845748,
-0.3731162548,
0.4229691029,
0.2541316152,
1.0161632299,
-0.119701907,
0.0995205566,
0.1050668061,
0.0040742573,
0.1287817955,
0.2428363264,
0.2005353868,
-0.3642730415,
-0.079679206,
0.1134931296,
-0.2830437124,
-0.335678786,
0.3455578387,
-0.1017288864,
0.5368952751,
0.1090376675,
0.1416432709,
-0.1509758532,
-0.185288474,
0.0773014799,
-0.070780091,
0.1030773222,
0.3541414738,
-0.0930450857,
0.1351346076,
0.2457795441,
0.1751324534,
0.0443398058,
-0.0947960988,
-0.279764384,
0.191700846,
0.3685204387,
0.0461871848,
0.0220657662,
0.0323536359,
0.3556630313,
-0.1849306375,
0.205039084,
-0.0305733252,
0.0079396451,
0.0666478723,
-0.0586210378,
-0.3365758359,
-0.6453829408,
0.2325167209,
-0.0009588738,
-0.1367515177,
0.1923418343,
0.1587267816,
-0.3540837169,
-0.2998668253,
-0.005748773,
0.2017253786,
0.1522040218,
0.2051188648,
0.2833249271,
-0.0867262706,
-0.3417101204,
-0.3844317198,
-0.046640411,
0.2829634845,
-0.4593487978,
0.078052409,
0.2093623281,
0.1067859381,
0.3750687242,
-0.0355154015,
-0.0439994782,
0.0425271429,
0.1307715178,
0.0788350254,
-0.1385990083,
0.159476012,
0.1490190029,
0.2470006347,
0.1999043524,
-0.2224675119,
-0.0522183515,
0.0016837198,
-0.0903742611,
-0.1905190051,
-0.1856554747,
0.1717607826,
0.122780256,
0.1018859744,
0.0458260253,
0.3413793445,
0.1714000404,
-0.2258879542,
0.1436648965,
-0.0075096581,
-0.1345750988,
-0.0918875337,
0.3624589443,
0.372572571,
-0.6563615799,
0.1040468812,
-0.0495636538,
0.1877676398,
0.1436999589,
-0.2795009613,
-0.2813878953,
0.4237989485,
-0.0935202166,
-0.1007727012,
0.3089181185,
-0.2708931565,
0.0815910101,
0.1968428344,
0.0673346817,
-0.2822614312,
0.2371013761,
-0.1123532876,
0.0422962159,
0.1624549329,
0.1141088828,
0.1663446426,
0.1164605319,
-0.0702251419,
-0.2196754515,
-0.1222945526,
-0.0431548283,
0.1172709763,
0.2665443718,
-0.1195797548,
0.1249020547,
0.5266056061,
0.3261623681,
-0.2632549703,
0.0764249414,
0.5432158113,
-0.3122918904,
0.0806592926,
0.1918925345,
-0.0554748699,
-0.0499001816,
0.1809134632,
-0.239194721,
0.4039112926,
0.0790574774,
-0.0053861486,
-0.2668074369,
-0.05302817,
-0.2360289097,
-0.4283643961,
0.2114924341,
0.046656061,
-0.0355188549,
0.2530861497,
0.052934926,
-0.090034768,
-0.2782234848,
-0.1389368922,
-0.8122122884,
0.2934662998,
-0.2860761583,
-0.0800811127,
-0.0875597671,
0.0202555042,
0.0639514253,
-0.1236340776,
-0.2867789865,
0.1009156108,
0.1873277426,
0.0372008197,
-0.0119494889,
0.1644939631,
0.12202999,
-0.2648279667,
0.3166706264,
0.3054232895,
0.1199404523,
0.0179686714,
-0.2019149363,
0.1108081341,
0.047732722,
0.3355243802,
0.0727866217,
0.2685894668,
0.1851827502,
0.1680479348,
-0.1562814564,
-0.1591640562,
0.3021561205,
0.4226676524,
0.181898281,
-0.1457033753,
0.0370392688,
0.0772159994,
0.4515741467,
0.1048432738,
-0.0922241807,
-0.1263715178,
-0.3054102957,
-0.0594788827,
0.4833451509,
-0.1791242361,
-0.0160226338,
0.2791059911,
-0.0161975473,
0.0771190077,
-0.1511851549,
-0.1175533831,
0.4446227849,
0.0723264888,
0.5178589821,
-0.025720587,
-0.1244163439,
-0.186818853,
-0.1908413172,
-0.2879426181,
-0.1234146729,
0.284741044,
-0.3124688864,
0.2768904269,
-0.4178830981,
-0.5642530322,
-0.2084295452,
0.4299706221,
-0.3480247557,
-0.3430694342,
-0.068008244,
0.0778863654,
-0.0229111258,
0.2500192523,
0.1072105244,
-0.0355423652,
0.2320451289,
0.0074740355,
-0.221677348,
-0.5956383348,
-0.4356403649,
0.0326633081,
0.2823260128,
0.0373753235,
0.1272295713,
-0.1569059193,
-0.0672415346,
-0.1452951729,
-0.4021958113,
0.3414440751,
-0.0869846046,
-0.0084635494,
0.1166531965,
0.4272187352,
-0.1959810108,
-0.0950585306,
0.3098011315,
0.1152016222,
-0.1476500183,
-0.0692660436,
-0.084672302,
-0.0623620301,
-0.0308235977,
-0.4383018911,
-0.1505850106,
-0.3273384273,
-0.1820001751,
0.1689834148,
0.1332358718,
0.3523069918,
0.2645772398,
0.2091481984,
0.3283596337,
0.0954611152,
-0.0965573341,
-0.1593371481,
0.2170185596,
-0.3185725212,
-0.3167464137,
0.1040421575,
-0.1413132399,
0.2305063754,
0.1135404631,
-0.1463303268,
0.068031691,
0.1159728244,
0.0200545825,
-0.10132052,
0.3257168531,
0.7469017506,
-0.0126238512,
-0.0016664611,
-0.0988115966,
0.0478255115,
0.0307381041,
-0.4368046522,
0.3473179638,
0.1777924001,
0.5277689695,
-0.070148021,
0.7515988946,
0.1148355007,
0.1166794449,
0.1321655512,
-0.0752610713,
0.0661863834,
-0.272472769,
-0.1884594262,
0.292780906,
0.0403269939,
-0.3030903637,
0.3898435831,
-0.1277018189,
-0.259891212,
-0.4387769997,
0.0750476867,
0.0015999969,
-0.2447388619,
-0.0376060195,
-0.375004977,
0.3839120865,
-0.0953027084,
0.399214685,
-0.0802457705,
-0.073142074,
0.0592005067,
0.2196860611,
-0.098245874,
0.1342238933,
-0.1730975062,
-0.2497494519,
-0.3993260264,
0.2461434305,
0.0012103891,
0.1436172575,
-0.2927531302,
0.0079170614,
0.2831737399,
0.0087450566,
0.5200991631,
-0.6470439434,
-0.0973823071,
0.3353391588,
0.1921804845,
-0.6571788192,
-0.1413873285,
-0.1876772195,
0.2771171927,
0.3285014331,
-0.0478471704,
-0.3322197497,
-0.1513581276,
0.2680895925,
0.0485687964,
-0.0990991369,
0.0515201502,
-0.2417584509,
-0.1433178782,
-0.3353154063,
-0.0657824501,
-0.0996943116,
0.1351074874,
0.1002396494,
0.2149960101,
0.0813032836,
0.0262184944,
-0.1448779553,
0.2050136477,
0.1226052344,
0.1383645535,
-0.2560669482,
0.1037121937,
0.4806474745,
-0.0265889727,
-0.1080245897,
0.1851126999,
-0.2079535574,
0.1726378053,
-0.2150923014,
0.1455086172,
0.1604102403,
-0.1206460595,
-0.2393639535,
0.036479231,
-0.2542997599,
-0.0464655384,
0.0528429002,
0.2087157369,
0.081001766,
-0.1274004281,
-0.4575787485,
0.5609187484,
0.1885293722,
-0.0729711279,
0.2990117073,
-0.0156652927,
-0.4306366444,
0.4052891731,
0.4329437912,
0.8635659814,
0.0299161691,
0.0139991231,
0.1714200377,
0.1671355665,
0.6254858375,
-0.5466775298,
0.2091643512,
-0.2812896073,
0.3198874891,
-0.0952550322,
0.0653772205,
0.3957331181,
0.1431851834,
-0.2221700996,
0.3536063433,
-0.0173850395,
0.0741585344,
0.0565966405,
0.3767171204,
0.1265330762,
-0.3078525364,
-0.1175291091,
0.1213966832,
-0.1823623627,
0.282468915,
-0.2498216778,
-0.0125389742,
-0.0145074334,
-0.2449238896,
-0.4106998742,
0.0911931768,
-0.2241830677,
0.4608557224,
-0.3423032165,
-0.4593053162,
0.2506963015,
0.2268088758,
0.2354612201,
0.3696658313,
-0.373550117,
0.2001676857,
0.0150043415,
-0.2361063957,
-0.0761885643,
0.0410973206,
0.3866564333,
-0.1922807992,
-0.392267853,
0.2118116468,
-0.1738399714,
-0.0978840888,
-0.2912859023,
-0.3177188337,
0.3188325465,
0.2502018809,
0.1240342706,
0.1725910157,
-0.0860564038,
-0.1338624209,
0.1198045388,
-0.2841424048,
0.0101037975,
0.0086888354,
0.0882756934,
-0.086818248,
0.0309087541,
0.2571510971,
0.187617898,
0.0172614269,
0.5952088237,
0.3785048425,
-0.2194746733,
-0.2659062147,
0.1786084622,
-0.2292431891,
-0.0606896169,
-0.2634163499,
0.1508059204,
0.0923175588,
-0.0571265519,
0.1029205695,
-0.0157441851,
-0.1409099549,
-0.0071758903,
-0.2680374682,
-0.0209585056,
0.0223001372,
-0.163809523,
-0.0015579847,
0.1883047223,
0.1332461685,
0.2853649259,
-0.2360227108,
-0.27911672,
-0.2388354242,
-0.0223193876,
0.0453208499,
0.413713336,
-0.0166400373,
-0.2360474467,
-0.038738545,
0.1452317834,
-0.230105862,
-0.245272398,
-0.1725503802,
-0.0468345471,
0.1290072948,
-0.0397848785,
-0.1874576509,
0.1358542144,
-0.3403597474,
-0.2925050855,
-0.2041294426,
-0.1316378266,
-0.1293067485,
-0.0242856704,
0.2222882509,
-0.1042087898,
0.3449907899,
-0.2878001034,
0.0879831091,
0.034875676,
0.2938313484,
0.0511716008,
0.2495484054,
0.4168607891,
-0.1764677018,
-0.5602070689,
0.1830452234,
-0.2211460471,
0.1743837148,
0.1633222252,
-0.0828021169,
-0.0480113775,
0.0299653579,
-0.0214734152,
0.026401462,
-0.0819887817,
-0.0302334055,
0.1355874985,
0.2045514137,
-0.2827225626,
0.1239571124,
0.4220982194,
-0.0330974758,
0.0961894989,
0.0262788963,
0.2615512311,
-0.0534214526,
0.1746624857,
0.0410569645,
0.0168640073,
-0.0434479043,
0.2639891207,
0.0946072638,
0.0811475441,
0.1160610095,
-0.1200714558,
0.046419926,
0.1310728341,
0.3746131361,
0.1071260795,
0.0660358295,
0.1008158401,
0.216569066,
-0.2253759801,
-0.1409866363,
0.3062991798,
-0.1027754843,
-0.0209083464,
0.0228770897,
-0.0645729229,
0.0748564154,
0.1480170339,
-0.0637498349,
-0.3180227578,
0.1386725008,
-0.0777270272,
-0.1830139905,
-0.6274287701,
-0.2322814912,
0.0546509698,
-0.0176587123,
0.0890894458,
-0.0836973041,
-0.1092470661,
0.2026976645,
-0.035025645,
-0.2306362838,
0.6797720194,
-0.0616486035,
0.0446974784,
-0.0551759414,
0.2520871758,
-0.213234365,
-0.0712455809,
-0.170553118,
-0.0018399562,
0.1578253806,
0.2109287828,
-0.4701038897,
-0.1439928114,
-0.0009009016,
0.0189440697,
-0.0591774844,
0.0006443821,
0.0630014166,
-0.0377971567,
0.2940510213,
0.1096708924,
-0.0898017213,
-0.1984425634,
0.2169163823,
-0.007181902,
-0.0200382136,
-0.24845469,
0.0045181345,
0.1042117402,
-0.1066087335,
0.1146248579,
-0.5152722597,
0.0413267612,
0.2594000697,
0.3884595931,
-0.1085284799,
-0.2229961902,
0.0836436301,
-0.3275438845,
0.5394181609,
0.084173657,
0.1905832291,
-0.1180475205,
-0.293014884,
-0.2950971127,
0.0054534362,
-0.1695943773,
-0.3680243194,
-0.1203949675,
0.3059184849,
0.3928039968,
0.2445940077,
0.1168807447,
-0.2535528839,
-0.129804939,
0.2650592625,
-0.1438631117,
-0.1589302421,
-0.0732823685,
0.0492415056,
-0.2251884341,
-0.1773683727,
0.0832060128,
-0.019431252,
0.0752257332,
-0.2276209742,
-0.1473571807,
0.3186531961,
-0.4347778857,
0.2595772445,
0.0258949678,
0.5472241044,
0.0291318949,
-0.0422727242,
-0.1655564606,
0.2228381783,
-0.0450003445,
0.4914211631,
0.0616208538,
0.228508994,
-0.215500325,
-0.3188501894,
-0.3452576101,
0.631701231,
0.0320963934,
-0.0550998375,
-0.2490800619,
-0.0586703122,
-0.2294992805,
0.2923284173,
-0.1192452237,
0.0662925616,
-0.036652118,
0.1867339313,
-0.3581700027,
-0.2367311567,
0.338419199,
-0.4211327136,
-0.3356799483,
-0.1897146255,
0.0682090595,
-0.1494336426,
0.2929887474,
-0.1057340801,
-0.0153544126,
0.2634702325,
-0.1352270991,
-0.0167238638,
0.00014365,
0.0598573945,
0.1573979855,
-0.2458987832,
-0.4044718742,
-0.1943297684,
-0.0603254735,
-0.3799313903,
-0.2492930144
]
|
https://github.com/huggingface/datasets/issues/1993 | How to load a dataset with load_from disk and save it again after doing transformations without changing the original? | Hi ! That looks like a bug, can you provide some code so that we can reproduce ?
It's not supposed to update the original dataset | I am using the latest datasets library. In my work, I first use **load_from_disk** to load a data set that contains 3.8Gb information. Then during my training process, I update that dataset object and add new elements and save it in a different place.
When I save the dataset with **save_to_disk**, the original dataset which is already in the disk also gets updated. I do not want to update it. How to prevent from this?
| 26 | How to load a dataset with load_from disk and save it again after doing transformations without changing the original?
I am using the latest datasets library. In my work, I first use **load_from_disk** to load a data set that contains 3.8Gb information. Then during my training process, I update that dataset object and add new elements and save it in a different place.
When I save the dataset with **save_to_disk**, the original dataset which is already in the disk also gets updated. I do not want to update it. How to prevent from this?
Hi ! That looks like a bug, can you provide some code so that we can reproduce ?
It's not supposed to update the original dataset | [
-0.2773599327,
-0.1424061358,
-0.0097127026,
0.2224388272,
0.2347680777,
0.1286466867,
-0.0314266682,
-0.0836327299,
-0.0445270352,
0.0425950624,
0.075677,
0.3508271873,
0.0656663328,
0.2150924355,
0.1894966364,
0.2755099237,
0.3028120697,
0.287147522,
-0.3395664692,
-0.1187535301,
-0.224780634,
-0.1271230131,
0.0034544617,
-0.2915345132,
-0.1811132729,
-0.2847265899,
-0.1051793545,
0.0112861227,
0.1192111149,
0.1282494366,
0.1472532898,
0.1170898676,
0.2598931193,
0.3208323717,
-0.0001200398,
0.0065588648,
-0.2820395231,
-0.0981556028,
-0.3171299398,
-0.1483111084,
0.0040692072,
-0.1686501652,
0.0125793694,
-0.3187102675,
0.0167110842,
-0.0577574261,
-0.1532115936,
-0.2047886103,
0.5439521074,
-0.2619330287,
0.107594572,
-0.0745563582,
-0.187593922,
0.0535860546,
-0.2637373507,
0.3224838674,
0.1635243446,
0.0492273457,
-0.0166151132,
0.357750684,
0.0076644314,
0.2626110911,
-0.2154628336,
-0.2576282918,
0.3756667078,
-0.0119469482,
0.1762690991,
-0.3532518446,
0.1566134393,
-0.0655310825,
0.73067981,
-0.4183008671,
-0.218370378,
-0.2186260372,
0.1446024477,
-0.1294231266,
0.1498940885,
0.2500553727,
-0.0096551413,
0.1328632683,
-0.5013387203,
-0.7865978479,
-0.0961474776,
0.0907198489,
0.0513803586,
-0.5484753251,
0.0074868756,
0.1316184402,
0.1669690758,
0.3084482551,
0.4444076419,
-0.2608911991,
-0.2427155226,
0.1032828763,
-0.290340215,
-0.3080196679,
-0.3247920573,
0.1850421131,
-0.0413482413,
0.2232469469,
0.0851406977,
-0.0896951258,
-0.2581597269,
0.1403543055,
0.1931943148,
0.2976646125,
0.1033944339,
0.207194671,
0.0640978366,
0.0867726803,
-0.2276675701,
-0.0555466637,
0.20996584,
0.1116869524,
0.6322052479,
-0.3062037528,
0.2605335712,
0.0181008801,
0.0575664267,
0.1173095033,
0.130124703,
-0.0381413139,
-0.1821729094,
0.3218333125,
0.1012515724,
0.1070649326,
0.1898790747,
0.2406489998,
-0.0559986159,
-0.131230399,
-0.1708797663,
-0.0795025527,
-0.302084893,
0.3862601519,
0.0738746449,
0.0053746747,
0.1103693098,
0.4839031398,
-0.4521855414,
-0.1569832563,
0.0093462383,
-0.1823614687,
0.3648670912,
0.1807543635,
0.1329002827,
0.1929759085,
-0.076700598,
-0.0259806067,
-0.0506211221,
0.703581214,
-0.3622857928,
-0.1167383566,
0.0317434445,
0.1288755238,
-0.0997812673,
0.1189192608,
-0.6574718952,
0.195701018,
0.1090384945,
-0.2294831127,
0.2326244712,
0.0644699186,
-0.3933760524,
-0.1955351233,
-0.0600749068,
0.2446707785,
-0.3517103195,
-0.1330958009,
0.1671471298,
-0.21639961,
-0.0562945195,
0.1603802443,
-0.2772349417,
0.2107775956,
-0.3416082859,
-0.3182986975,
0.5734580159,
0.1237904206,
-0.4386878908,
0.1376537681,
-0.0473179966,
-0.0854984671,
-0.0338461138,
0.5759631991,
0.0887063146,
-0.1218515038,
-0.4468071163,
0.2835900187,
0.0914308503,
0.0993331671,
-0.0000940634,
-0.1719220877,
0.2446640432,
-0.3138799369,
-0.1098825559,
0.4869319201,
0.2913514078,
0.4426919818,
0.334646374,
0.0546468161,
0.1226764545,
0.4205861688,
-0.1444273144,
0.0363452137,
-0.1889340132,
0.1604402065,
-0.6050275564,
-0.0283060186,
0.1515420228,
-0.5714520216,
0.4265670478,
-0.0966279879,
-0.0594581477,
-0.2061413974,
-0.0825064108,
0.0565846972,
-0.0742595419,
0.0540459976,
-0.0702317655,
-0.1691202372,
-0.2333438098,
0.456592083,
-0.0745810866,
0.1019803286,
-0.6434776783,
0.4120724797,
0.1448137611,
-0.1876071393,
-0.2897028923,
-0.0770047233,
0.2022824734,
0.0158427823,
-0.1604729891,
0.3794603348,
0.1969829947,
0.3693661094,
-0.0724357069,
0.1969145834,
0.0275860857,
-0.03662977,
0.0015109699,
-0.1320199668,
0.1783736944,
-0.0849592537,
-0.1314107776,
-0.0251699276,
-0.1762410551,
0.0567239672,
0.069345735,
-0.1900814921,
0.006539701,
-0.322676152,
-0.0129020093,
-0.2311117649,
-0.2205688059,
0.1944511384,
0.3617493808,
0.1074927822,
-0.1966824681,
-0.1728538573,
0.2807906866,
-0.2587949038,
-0.046454668,
0.04439326,
-0.2743505836,
0.0095040658,
-0.126094535,
0.5707715154,
0.1891009361,
0.1061207056,
0.0777843669,
0.1742013544,
0.1364917308,
-0.0232195966,
0.0245525353,
0.0353945792,
0.5409007072,
0.2572125196,
-0.0064347847,
0.0857305303,
-0.1593878418,
0.4665907323,
0.1098956391,
-0.1207866445,
-0.3045049906,
0.1523126364,
-0.1394733638,
0.0934548676,
-0.4907520413,
-0.085854955,
-0.2307415754,
0.1629550308,
-0.253011018,
0.7551638484,
0.2067698687,
0.1947493553,
0.1520791054,
0.0233588722,
-0.088505432,
-0.4377728999,
-0.0893530548,
0.1271412671,
-0.0993077382,
-0.0616394021,
0.0731258318,
-0.0676448718,
0.391498208,
-0.0824565664,
-0.0544499233,
-0.4722399116,
0.0678149834,
-0.0471549295,
0.0970693007,
0.1179436892,
-0.17211169,
0.1620835662,
-0.402690798,
-0.0154979546,
-0.1130088717,
-0.4427280426,
-0.2376412898,
0.0469757989,
0.1358091533,
0.2856688201,
-0.0207174532,
-0.1760309041,
0.0313204192,
-0.024033349,
-0.164477542,
-0.179878965,
0.0381253064,
0.0707306042,
0.178548798,
-0.2493127584,
0.2252116352,
0.0366106778,
-0.2901350856,
-0.5891330838,
0.3810377419,
0.1769791245,
-0.2777746916,
0.2593784034,
-0.1830546856,
-0.0385803692,
0.3359547555,
-0.6108108163,
-0.0890947059,
0.0473069362,
0.0712169558,
-0.4067152739,
0.326680094,
0.3233914971,
-0.1105937883,
0.0090249348,
-0.1059060618,
-0.2592622638,
0.0587808453,
0.0994871333,
0.4200101197,
-0.202308923,
0.2221486866,
-0.0814594924,
0.254273504,
-0.0070600715,
-0.1169081181,
0.3640682101,
0.113461934,
0.5133029222,
-0.2445792705,
-0.1410066187,
-0.5643543005,
-0.1771261841,
-0.2818342447,
-0.0114475191,
-0.0227354951,
-0.0442443527,
-0.1466463804,
-0.2983010411,
-0.2498426288,
-0.1560131013,
-0.000416379,
-0.2736836076,
0.3052912056,
0.1021624953,
0.2262858599,
0.1891043484,
-0.2032569498,
-0.0671014115,
0.2009995133,
0.314853847,
0.0471926108,
-0.3226696849,
-0.0306130201,
-0.1401811838,
0.1583726108,
-0.208939597,
-0.0048105107,
0.1612967104,
-0.0642388389,
0.0978770629,
0.2093059868,
0.8259218335,
0.2282083631,
0.1617145985,
0.0667234138,
-0.3872631788,
-0.2855739892,
-0.0050815786,
0.0198308267,
-0.2780984342,
-0.08809302,
0.6831376553,
0.1787527055,
-0.0507894009,
-0.2957939208,
0.4659901857,
-0.3318035305,
-0.3347010016,
-0.260348618,
-0.199174881,
-0.5212273002,
-0.1314110905,
-0.2619122565,
-0.0284723788,
-0.1773986816,
0.1137883291,
-0.1871512234,
-0.0758932456,
0.0513755195,
-0.179871425,
0.3351958096,
0.3329256177,
0.0540518165,
0.082394287,
0.1873525083,
0.1717302054,
0.3534222543,
-0.0996236056,
-0.0959057361,
-0.0483043194,
0.068113707,
0.1413039863,
0.0803877562,
0.0522795357,
0.0050396193,
-0.0257189032,
-0.2154927701,
-0.3657270968,
-0.085214898,
-0.0878768116,
-0.1379947364,
-0.2146566957,
-0.4565047622,
0.5085779428,
-0.0864709243,
-0.08469522,
-0.0616627783,
-0.0977538601,
-0.2706188262,
0.107446067,
0.1182118133,
0.8301042914,
0.1662537307,
0.2188904583,
0.1812034696,
-0.2468580753,
0.0367079675,
0.028986644,
-0.0480848476,
-0.3911786377,
-0.3459461033,
-0.1515959501,
-0.1716965735,
0.0086909709,
0.3013532758,
-0.3460031748,
0.3065207005,
-0.278655082,
-0.0542788506,
-0.1268516928,
-0.0232127644,
-0.1616661698,
-0.130897671,
-0.1627980471,
0.0030435778,
-0.0186125804,
0.0688089505,
-0.0729741305,
0.0433280803,
0.1778716296,
-0.1023885459,
0.3078854978,
0.1381433755,
-0.0280989818,
0.2491618693,
-0.1203391254,
-0.4506576955,
-0.0506094173,
0.5732134581,
0.4336514175,
0.053010799,
0.0687487051,
-0.0989169329,
-0.0141946012,
0.390299201,
-0.1978022158,
-0.1549126804,
0.4320684969,
0.1253274977,
-0.0773230791,
0.0669241175,
-0.0534173362,
-0.1615176201,
-0.0730298236,
-0.0121715236,
0.0610186122,
-0.3188638091,
0.0215415936,
0.238542214,
0.3357429206,
-0.106353052,
0.0380113907,
0.004773851,
-0.0055089309,
0.2677952945,
0.0356958956,
-0.2979890406,
0.0134074744,
0.5746380091,
0.2181511521,
-0.0201750379,
0.4256411791,
-0.333548665,
0.1248633042,
-0.2270947695,
0.5075287223,
0.1834753454,
-0.2038391829,
0.204584375,
0.0383670069,
0.4741450846,
-0.0272538103,
-0.0167224593,
0.0738130882,
-0.0079367766,
0.1543699205,
-0.0486601032,
-0.3517206609,
0.0910364687,
0.228633821,
0.3515548408,
0.345895499,
-0.1389694959,
0.0248693563,
0.3707377017,
-0.2032515109,
0.1789879203,
0.2704665959,
0.2227462977,
0.2163433433,
0.2249100804,
-0.3069208562,
-0.3214676976,
-0.0353056379,
-0.1933948994,
-0.2613627613,
-0.1477647126,
-0.0689215809,
0.1421385258,
-0.0735851303,
0.2744121552,
-0.2740538716,
-0.4286926985,
0.1984904855,
-0.1260866076,
0.1044978425,
0.1898787916,
0.2151739597,
0.2136213034,
0.1381161958,
0.463435173,
-0.2713992596,
-0.1352325827,
-0.2096986771,
0.1733742207,
-0.0220644493,
-0.0064738221,
0.1258608699,
-0.1178692356,
-0.624211967,
-0.0673954412,
0.5685312152,
0.1167621017,
-0.0426341705,
-0.228073135,
0.0550828911,
0.1555532515,
0.2226058394,
0.1180747226,
-0.4023565352,
-0.3365199566,
0.3168421984,
0.1549125314,
-0.0792565942,
-0.1912566274,
0.2755455971,
-0.1716841906,
-0.3012566864,
0.1850246489,
0.1411781609,
-0.0526255369,
-0.3393751979,
0.0854594335,
0.2024498582,
-0.1482756883,
0.402292043,
0.6510440707,
-0.2748962641,
0.02343891,
0.1474157572,
0.3377689719,
-0.1483324468,
0.4305062294,
-0.0011164823,
0.4770655334,
-0.0052317143,
0.0808772519,
-0.1037395895,
-0.4036500752,
0.0769738704,
0.1380402446,
-0.2588015497,
0.0405354165,
-0.0300863329,
-0.1536857039,
0.1886299551,
-0.3479962647,
-0.1480425149,
0.371460855,
-0.2411905825,
0.1074003875,
-0.0134342313,
-0.1359700114,
0.2627212107,
0.245537892,
-0.0099923182,
-0.2409634143,
0.379596591,
0.0855145231,
-0.1817889214,
0.1468252093,
0.0894482359,
0.203043133,
-0.1944677085,
-0.1617190242,
0.0693567172,
-0.1237477139,
0.0871068239,
0.0874504447,
0.0389343053,
-0.1845511496,
0.1138074175,
0.2477377504,
0.2041705549,
0.2740830183,
-0.0373132415,
0.129121244,
0.1125141382,
-0.0035212163,
0.0093156826,
0.2051872611,
-0.0007487879,
0.0038971787,
0.3048615456,
0.094767116,
0.38232705,
-0.4848355353,
0.259547174,
0.2937277257,
-0.0746514648,
-0.2495840192,
0.0423015431,
0.1689218134,
0.0155461011,
0.552826941,
-0.2686410546,
0.0056019058,
0.0508065745,
0.038175106,
0.0514431819,
0.4367138147,
0.2386618555,
-0.449611634,
-0.2709538043,
-0.2319020331,
-0.5596633554,
0.0184254553,
0.3477914929,
0.313118577,
-0.2493396401,
0.1025706381,
0.0763873532,
0.1652165204,
0.0482026339,
-0.1767880321,
0.0744335204,
0.3412542939,
0.1767935902,
-0.253485769,
-0.0234436393,
0.2920182645,
-0.1093694568,
-0.25149405,
0.1846387833,
0.0725315884,
-0.0073190029,
0.178551659,
0.1702842414,
-0.2235814333,
0.4020434618,
0.6338938475,
0.0853700861,
0.0235495083,
-0.1998800039,
0.2453511655,
0.320682466,
-0.0403757095,
-0.1977474242,
-0.0849017501,
0.0871069953,
0.1833111793,
-0.3971989453,
0.3609266877,
-0.2117162496,
-0.112946108,
0.1277287602,
0.0651458055,
-0.5358834863,
0.0491106026,
0.0694132745,
0.1766889095,
0.1368402243,
0.3822602332,
0.0120540559,
0.345138818,
-0.1944079101,
-0.1288729757,
0.3826499581,
-0.1838702559,
-0.3120287657,
-0.1820346117,
0.3298673332,
-0.0515801646,
-0.1072216704,
-0.3974067271,
0.1122053489,
0.4550142288,
-0.2239275277,
0.0127509292,
0.5110883117,
-0.2035416067,
0.1770402938,
0.1363916993,
0.5885226727,
0.1271755397,
-0.3351359665,
-0.0040454818,
-0.3007531166
]
|
https://github.com/huggingface/datasets/issues/1993 | How to load a dataset with load_from disk and save it again after doing transformations without changing the original? | Hi, I experimented with RAG.
Actually, you can run the [use_own_knowldge_dataset.py](https://github.com/shamanez/transformers/blob/rag-end-to-end-retrieval/examples/research_projects/rag/use_own_knowledge_dataset.py#L80). In the 80 you can save the dataset object to the disk with save_to_disk. Then in order to compute the embeddings in this use **load_from_disk**.
Then finally save it. You can see the original dataset object (CSV after splitting also will be changed)
One more thing- when I save the dataset object with **save_to_disk** it name the arrow file with cache.... rather than using dataset. arrow. Can you add a variable that we can feed a name to save_to_disk function? | I am using the latest datasets library. In my work, I first use **load_from_disk** to load a data set that contains 3.8Gb information. Then during my training process, I update that dataset object and add new elements and save it in a different place.
When I save the dataset with **save_to_disk**, the original dataset which is already in the disk also gets updated. I do not want to update it. How to prevent from this?
| 91 | How to load a dataset with load_from disk and save it again after doing transformations without changing the original?
I am using the latest datasets library. In my work, I first use **load_from_disk** to load a data set that contains 3.8Gb information. Then during my training process, I update that dataset object and add new elements and save it in a different place.
When I save the dataset with **save_to_disk**, the original dataset which is already in the disk also gets updated. I do not want to update it. How to prevent from this?
Hi, I experimented with RAG.
Actually, you can run the [use_own_knowldge_dataset.py](https://github.com/shamanez/transformers/blob/rag-end-to-end-retrieval/examples/research_projects/rag/use_own_knowledge_dataset.py#L80). In the 80 you can save the dataset object to the disk with save_to_disk. Then in order to compute the embeddings in this use **load_from_disk**.
Then finally save it. You can see the original dataset object (CSV after splitting also will be changed)
One more thing- when I save the dataset object with **save_to_disk** it name the arrow file with cache.... rather than using dataset. arrow. Can you add a variable that we can feed a name to save_to_disk function? | [
-0.2981738746,
-0.0828650892,
0.026727628,
0.1493992209,
0.3067895174,
0.1228003353,
-0.056206774,
-0.0614769422,
0.0445273146,
0.0541939661,
-0.0652613267,
0.373824805,
0.0215632487,
0.1011980772,
0.1474012882,
0.2532072067,
0.1987997591,
0.2516978383,
-0.2688195407,
-0.0971939489,
-0.2197249532,
-0.2309300303,
0.0529336184,
-0.3456068039,
-0.2162120193,
-0.2678651512,
-0.1700970232,
0.0145697892,
0.1032242253,
-0.0398215726,
0.1639600545,
0.1300251037,
0.3564485908,
0.2765147686,
-0.0001222565,
0.0449469239,
-0.3267863691,
-0.1661964208,
-0.3974144459,
-0.1439908296,
0.1779916286,
-0.2638607919,
0.0647074729,
-0.3373178542,
-0.044642739,
-0.1765089482,
-0.1076053977,
-0.2636475563,
0.6995683312,
-0.3083577752,
0.0656188875,
-0.0540520251,
-0.242259115,
0.0614363104,
-0.0760421902,
0.2449965924,
0.1191360131,
0.1519679278,
-0.0484970808,
0.222602725,
-0.1404743642,
0.2548884451,
-0.206185624,
-0.1893692315,
0.3967930675,
-0.0019805157,
0.0755403489,
-0.3313625455,
0.078287378,
-0.0002815274,
0.7876373529,
-0.4693835378,
-0.3323039114,
-0.2304031998,
0.2201096565,
-0.0707609132,
0.0433409587,
0.2069169283,
0.0135486741,
0.2221799642,
-0.4472396076,
-0.8105935454,
-0.1399761587,
0.148846522,
0.081921421,
-0.4945167303,
0.0238876827,
0.1483843327,
0.2077623159,
0.2897449434,
0.3728309274,
-0.2549823523,
-0.174267441,
0.1039848253,
-0.304441005,
-0.2441856563,
-0.2922514975,
0.0569521487,
-0.0547050759,
0.3812344968,
0.134929359,
-0.0329645649,
-0.2412495464,
0.1737682819,
0.1737946868,
0.2897123992,
0.1109259129,
0.1706048548,
0.137961477,
0.0049736253,
-0.2106242627,
-0.1055164337,
0.1187184826,
0.1315823197,
0.5387067199,
-0.216119051,
0.210535422,
0.0456550457,
0.1234001517,
0.0126177771,
0.0549341179,
-0.0481305085,
-0.1775923818,
0.3871257603,
0.0820183158,
0.1812814772,
0.1506491452,
0.2366102785,
0.0228762943,
-0.1150492877,
-0.126182884,
-0.034810748,
-0.2900650799,
0.3913138211,
0.200962618,
-0.0995992348,
0.1868815571,
0.406493634,
-0.434612304,
-0.1599065065,
0.014104466,
-0.2374428213,
0.5390254855,
0.1750428081,
0.0522037372,
0.2025617361,
0.0051056952,
-0.0455212891,
-0.16679959,
0.5195535421,
-0.2719987929,
-0.1811146438,
0.1025095284,
0.0902322009,
-0.1660820842,
0.211394459,
-0.4340533912,
0.1076641455,
0.0573430248,
-0.129347682,
0.1663852334,
0.0311018843,
-0.3366868794,
-0.2785730362,
0.0628455058,
0.3072366416,
-0.421838671,
-0.1641578674,
0.0618917644,
-0.0940393806,
-0.2057060152,
0.2003053874,
-0.2843593061,
0.2975260913,
-0.2760421038,
-0.2422117144,
0.5749605894,
-0.0287338328,
-0.4812774956,
0.1532567143,
0.0253596324,
0.0056968955,
0.0016571047,
0.6175723076,
0.3191412389,
-0.1399005949,
-0.4540525377,
0.3999415636,
0.1578349322,
0.1049263328,
0.0218710192,
-0.2957141399,
0.218394056,
-0.286896497,
-0.187124595,
0.3673588932,
0.2825941741,
0.2646552622,
0.3615920544,
0.023433432,
0.1554547548,
0.3602967858,
0.022047203,
0.0340865143,
-0.1074189991,
0.0527727343,
-0.6752665639,
-0.0008339949,
0.1554767638,
-0.5374923348,
0.1736090183,
-0.1934854835,
-0.0924268737,
-0.1653457284,
-0.0885925964,
-0.0135689853,
-0.0897684395,
-0.0308936052,
-0.0441638045,
-0.2119159997,
-0.2746409476,
0.420181185,
-0.0376424603,
0.1762324125,
-0.620796144,
0.4170428216,
0.1973152906,
-0.2141879499,
-0.1617541909,
-0.0837891176,
0.2295796275,
0.0185934585,
-0.0512566306,
0.3639731109,
0.1689337939,
0.3720763624,
0.0581999049,
0.1233945265,
0.0281125568,
-0.1681798249,
0.2201289386,
-0.0435152203,
0.1642445922,
-0.1232946441,
-0.2530218661,
0.0886411369,
-0.1890543103,
0.1372635216,
0.0867500901,
-0.1663255244,
-0.0118460236,
-0.3778167367,
-0.0842532814,
-0.2564433217,
-0.2976900339,
0.1848402619,
0.4458978474,
0.06325344,
0.0249879453,
-0.1737430692,
0.3163312376,
-0.1756475717,
0.0119173033,
0.0152702266,
-0.3919639885,
-0.0695015565,
-0.1718242466,
0.488899976,
0.1010779515,
0.0891602933,
0.0631393939,
0.1061020494,
0.1201622486,
0.0404634885,
0.0341840535,
0.018937327,
0.5688117146,
0.3238119781,
0.0262622833,
0.0392554887,
-0.1586348414,
0.4841209948,
0.1311213523,
-0.1580862701,
-0.2029996216,
0.0602509491,
-0.1202483699,
0.2065155655,
-0.4876646399,
-0.1865927726,
-0.3327080905,
0.1092468202,
-0.256514132,
0.6586429477,
0.2108454108,
0.2717782557,
0.158767879,
0.0456166565,
-0.1248293966,
-0.4218959808,
-0.0536407307,
-0.0312548131,
0.0374602601,
-0.0909013599,
-0.0181662049,
0.0350253806,
0.4715486765,
0.1138014719,
-0.03066919,
-0.5500054359,
0.0920293033,
-0.0775487944,
0.2022334337,
0.0827579349,
-0.1859748513,
0.1372918785,
-0.236660108,
-0.0745643377,
-0.110895358,
-0.4580129981,
-0.176192984,
-0.0046439138,
0.0675836802,
0.3397306204,
0.0321200192,
-0.3095199764,
-0.0657436773,
-0.0772383735,
-0.1141659692,
-0.1819244325,
-0.0122262007,
-0.0531609319,
0.1659827679,
-0.2359537929,
0.23220478,
0.005327893,
-0.2006024718,
-0.4661620259,
0.4412538111,
0.1245173141,
-0.1237185448,
0.2641140521,
-0.2815725803,
-0.165634945,
0.4785481095,
-0.524648428,
-0.1548045129,
0.0558007807,
0.1381168664,
-0.3403528333,
0.4552119672,
0.3021211624,
0.047657527,
0.0140214963,
-0.072477147,
-0.2228310704,
0.0957026631,
0.0429992862,
0.4033215344,
-0.1240114048,
0.1896509975,
-0.0526101217,
0.4766649902,
0.048253417,
-0.1304225922,
0.2802928388,
0.1950444281,
0.472826153,
-0.1371493936,
-0.1203952879,
-0.4867362976,
-0.2577696145,
-0.2264501303,
-0.0197652392,
0.0007065286,
0.0517285615,
-0.0943003297,
-0.2863279283,
-0.1904704869,
-0.2041419595,
0.0761580989,
-0.2113740593,
0.313575387,
0.0572802164,
0.183583796,
0.122152105,
-0.1776897162,
0.0991703495,
0.1698619574,
0.4022676349,
0.0150108691,
-0.3136447966,
0.0082833301,
-0.1507182866,
0.1457837969,
-0.2008711994,
-0.1088744253,
0.2638158798,
-0.0960787907,
0.082612589,
0.2346357256,
0.8441631794,
0.094590731,
0.093668744,
0.0452635102,
-0.2911585569,
-0.2852756679,
0.0557535663,
0.0138807418,
-0.2369900644,
-0.1452256441,
0.6316407919,
0.0028977301,
-0.0583516248,
-0.1630969644,
0.5997645259,
-0.4410639107,
-0.3710057735,
-0.1498326808,
-0.142398566,
-0.5282942057,
-0.2274989337,
-0.278373301,
-0.1651142985,
-0.1657163203,
0.1216927841,
-0.3377402127,
-0.0797513127,
0.1186226085,
-0.1386128366,
0.3896144629,
0.2384509891,
0.055058796,
0.1810156703,
0.3151733279,
0.2113416344,
0.4770444036,
-0.2193967253,
-0.3072482944,
-0.1956390589,
0.1561986804,
0.1901346296,
0.1089461967,
0.0555662811,
0.0437806472,
-0.0478343703,
-0.1942572743,
-0.4450426996,
-0.1347372979,
-0.1656711251,
-0.0990397856,
-0.1916394532,
-0.4690859914,
0.6322374344,
-0.173989445,
-0.1316200793,
-0.1235204414,
-0.0665299967,
-0.2540264428,
0.3347471058,
0.1608248651,
0.9694904685,
0.0472938307,
0.1549808979,
0.1151880473,
-0.3309181929,
0.1146972105,
-0.0952975079,
-0.0556070693,
-0.4986627996,
-0.2365258038,
-0.1501408964,
-0.1499622017,
0.0369532481,
0.4310849607,
-0.3788289726,
0.3566516638,
-0.320109129,
-0.0296786558,
-0.1564430296,
0.1767745912,
-0.1408026367,
-0.2388235927,
-0.1166878045,
-0.0169759747,
0.0095412135,
0.1132640615,
0.0370528996,
0.0269384049,
0.1899424642,
-0.019800853,
0.2504062057,
0.1620724201,
-0.0853406414,
0.3151873052,
-0.1668690443,
-0.5314211249,
-0.0107573988,
0.5266742706,
0.5019901395,
0.0503389798,
0.0927918926,
-0.0129765207,
0.0295861084,
0.2790664136,
-0.1464172006,
-0.1045440361,
0.4724716842,
0.1752949655,
-0.2453790754,
0.207908541,
-0.0959704295,
-0.1067542881,
0.0770221502,
-0.0453322604,
0.2414695323,
-0.3650998175,
0.0446831882,
0.267370671,
0.2766036987,
-0.0850387812,
-0.0106885247,
0.0355996415,
0.0274110343,
0.2962163985,
0.021650875,
-0.2268107533,
0.073902525,
0.4579524398,
0.2298098058,
0.0274650939,
0.4529787004,
-0.3027491868,
0.0437543504,
-0.2348896712,
0.3578218222,
0.1558244228,
-0.2728921473,
0.1640938669,
0.1499302834,
0.3521018326,
-0.0766547248,
-0.1872917563,
0.0960826427,
0.0095182685,
-0.0317108408,
-0.0927924812,
-0.3228533864,
0.150191918,
0.3122368157,
0.3856065571,
0.2359103262,
-0.2864778638,
-0.0425243899,
0.4829114676,
-0.2021657526,
0.2236266434,
0.2858694792,
0.2984408736,
0.0155727277,
0.1222398654,
-0.263071686,
-0.3910294771,
-0.0439399444,
-0.2537715435,
-0.2928432524,
-0.1167141423,
-0.0936504602,
0.1613434553,
-0.1719065905,
0.2861395776,
-0.3794547319,
-0.4428187907,
0.2977696359,
-0.2330526263,
0.1779194921,
0.2634783685,
0.2481666207,
0.229486987,
0.1904305518,
0.3597934246,
-0.322896421,
-0.0372621045,
-0.2004568279,
0.176751107,
0.019368697,
0.0437841043,
-0.0080935778,
-0.1049269736,
-0.500638783,
-0.0623634011,
0.5708613396,
0.0377840176,
0.0752919316,
-0.1121589467,
-0.0733085051,
0.0764946342,
0.2585808635,
0.1424664557,
-0.31663993,
-0.3115602434,
0.3152827621,
0.1083468795,
-0.1660611331,
-0.1455035359,
0.2591179311,
-0.3066039383,
-0.2280289829,
0.1627317667,
0.0407927558,
0.0030275439,
-0.3377047479,
0.0188240316,
0.3602540791,
-0.1565861553,
0.3047995865,
0.4423649311,
-0.0967153385,
-0.0677862018,
0.1052056924,
0.3428692818,
0.0583967455,
0.2408365756,
-0.0606637597,
0.3778834641,
-0.0158731528,
0.0611627772,
-0.0311945677,
-0.4003717899,
0.0675602481,
0.2207543403,
-0.2321503162,
0.0245229807,
0.0097705862,
0.0145139005,
0.2708434761,
-0.3510391116,
-0.1519547105,
0.3862371743,
-0.0674808696,
0.0649071485,
0.050135348,
-0.1028005108,
0.220330596,
0.2604192793,
-0.0059281643,
-0.3765154183,
0.382373333,
0.1547003984,
-0.0371920876,
0.1426367313,
0.0961539894,
0.1613835245,
-0.0567561276,
-0.2217995375,
-0.0699081123,
0.0152477054,
0.1601682156,
0.045415882,
-0.0462475233,
-0.1148170009,
0.0529403239,
0.1100774482,
0.33444646,
0.3077133,
-0.0333200172,
0.088646464,
-0.0147227002,
-0.1016333178,
0.0101880291,
0.2018377334,
0.000448887,
0.0180066824,
0.1105644628,
0.0184146892,
0.337485522,
-0.3615120649,
0.2868886292,
0.3628126979,
-0.1820758879,
-0.1669217646,
0.0322595499,
0.131151095,
-0.0288601667,
0.6571771502,
-0.3129006326,
-0.0014364816,
0.1126715466,
0.0295943059,
-0.1300789863,
0.2919332683,
0.3886235356,
-0.4222635329,
-0.2638187706,
-0.1415784657,
-0.5935501456,
-0.0703666434,
0.2431906313,
0.1278578192,
-0.233816281,
0.1324103624,
0.207968086,
0.2505530119,
-0.0467725284,
-0.2302669585,
0.1695550531,
0.3301642537,
0.0832539871,
-0.23906672,
-0.0924554318,
0.4059372246,
-0.079902038,
-0.3620832562,
0.0415074825,
0.189649716,
-0.057338912,
0.2455222309,
0.1919704378,
0.0094556147,
0.3001290858,
0.6162530184,
-0.0190494955,
-0.0020516024,
-0.204789415,
0.2092383355,
0.4031696618,
0.022274429,
-0.1401595175,
-0.0994514152,
0.1238332018,
0.2696533799,
-0.4371400177,
0.3272205591,
-0.2485332489,
-0.1709991097,
0.0642754585,
0.0851401091,
-0.4315862656,
0.050965175,
0.1431972235,
0.2185951173,
0.1820221692,
0.3784556389,
-0.068585515,
0.3610673249,
-0.1881099194,
-0.1009037495,
0.4753728807,
-0.1566340029,
-0.2016952932,
-0.0870269462,
0.4287044108,
-0.0821657255,
0.0284779444,
-0.5293525457,
0.0917173177,
0.4609173238,
-0.2307269573,
-0.0510533601,
0.4078030586,
-0.0736483112,
0.1740235388,
0.0477650166,
0.4229540825,
0.1043647006,
-0.3273549378,
-0.174510479,
-0.3433351219
]
|
https://github.com/huggingface/datasets/issues/1993 | How to load a dataset with load_from disk and save it again after doing transformations without changing the original? | @lhoestq I also found that cache in tmp directory gets updated after transformations. This is really problematic when using datasets interactively. Let's say we use the shards function to a dataset loaded with csv, atm when we do transformations to shards and combine them it updates the original csv cache. | I am using the latest datasets library. In my work, I first use **load_from_disk** to load a data set that contains 3.8Gb information. Then during my training process, I update that dataset object and add new elements and save it in a different place.
When I save the dataset with **save_to_disk**, the original dataset which is already in the disk also gets updated. I do not want to update it. How to prevent from this?
| 50 | How to load a dataset with load_from disk and save it again after doing transformations without changing the original?
I am using the latest datasets library. In my work, I first use **load_from_disk** to load a data set that contains 3.8Gb information. Then during my training process, I update that dataset object and add new elements and save it in a different place.
When I save the dataset with **save_to_disk**, the original dataset which is already in the disk also gets updated. I do not want to update it. How to prevent from this?
@lhoestq I also found that cache in tmp directory gets updated after transformations. This is really problematic when using datasets interactively. Let's say we use the shards function to a dataset loaded with csv, atm when we do transformations to shards and combine them it updates the original csv cache. | [
-0.3775414824,
-0.1095238999,
0.001331388,
0.2857869565,
0.2583921552,
0.1318970025,
-0.0723696798,
-0.0919264182,
-0.0414135642,
-0.0703071728,
-0.0545120724,
0.2699585855,
0.0232360195,
0.0732332394,
0.1965225935,
0.2476300597,
0.2620952725,
0.2115163654,
-0.4262392223,
-0.0511153378,
-0.2185808718,
-0.1405914426,
-0.0047839251,
-0.2865780294,
-0.2252599001,
-0.3567643464,
-0.0927875564,
0.0716758296,
0.2163682431,
0.0895130634,
0.1970446259,
0.1367613673,
0.2189594954,
0.3962475657,
-0.0001211941,
0.0404281877,
-0.3316934407,
-0.1156463623,
-0.3154460192,
-0.0537975505,
0.0001288081,
-0.2120254487,
0.0525792502,
-0.2816854417,
-0.0468885936,
0.0064461883,
-0.1075409651,
-0.3362382948,
0.5601174235,
-0.2909074724,
0.0684704334,
-0.1258435994,
-0.3435740769,
0.1475198716,
-0.2772859931,
0.3350456655,
0.1380087733,
-0.1039553732,
-0.0801336169,
0.3443602622,
0.0155065358,
0.2434511036,
-0.2749490142,
-0.1867040694,
0.3630914688,
-0.0399342477,
0.0585349947,
-0.25517869,
0.2135362923,
-0.0502433851,
0.731131196,
-0.4320099354,
-0.2579138279,
-0.2524375916,
0.0672599226,
-0.0608988218,
0.1881187856,
0.2648539245,
0.1020966172,
0.177375257,
-0.667437017,
-0.7337231636,
-0.0253955498,
0.1337726265,
-0.0163028855,
-0.5745208859,
0.0006099135,
0.148791045,
0.0624436848,
0.239105165,
0.4866165519,
-0.2371123433,
-0.3087270558,
0.1965989918,
-0.3022063673,
-0.1864705831,
-0.2256158888,
0.239522323,
-0.1068107188,
0.1615791917,
0.0734234676,
-0.0433908291,
-0.3852363229,
0.12986736,
0.1477089375,
0.3210478127,
0.1508118808,
0.2113259584,
0.1089459434,
-0.1171295419,
-0.2213821709,
-0.0770059675,
0.2559016645,
0.0755557567,
0.6239587665,
-0.2735369205,
0.1777047217,
0.0221851803,
0.1790606529,
0.0160736982,
0.148289308,
-0.0388493985,
-0.1566103995,
0.3401576281,
0.0454191118,
0.1725090444,
0.1815034002,
0.1542690098,
-0.0600081012,
-0.1481688023,
-0.1722120494,
-0.1459452063,
-0.295217663,
0.4529230893,
0.1697225124,
-0.0070342179,
0.0887550265,
0.4451131821,
-0.4322245717,
-0.1200901344,
-0.0233444013,
-0.1521679014,
0.4138382077,
0.232397005,
0.1351475865,
0.2169104069,
-0.0307578854,
0.0287326053,
-0.0869794041,
0.6420640349,
-0.3159516454,
-0.1536168903,
0.2198777944,
0.0855470151,
-0.2015257329,
0.1320276707,
-0.6194604635,
0.1727586091,
0.2716605663,
-0.1368694156,
0.1567058265,
0.0461920016,
-0.3810899556,
-0.2422453314,
-0.0971095264,
0.3406148255,
-0.4246287346,
-0.2163509578,
0.2547136247,
-0.1289500147,
-0.2241908759,
0.2647134066,
-0.2679210305,
0.2254050523,
-0.2347068787,
-0.3467521667,
0.5462708473,
0.0552272685,
-0.39888376,
0.0837444216,
0.0544949137,
-0.0453632511,
-0.0010168505,
0.5464831591,
0.1674202979,
-0.1307810247,
-0.516481638,
0.3973065317,
0.1192879602,
0.1762743443,
0.0391114168,
-0.1514858305,
0.2610328794,
-0.2685463428,
-0.0825342163,
0.4303972423,
0.2913789153,
0.2922709584,
0.214286983,
0.0226374716,
0.189432472,
0.3739202917,
-0.184254989,
0.1289747506,
-0.1220934242,
0.1327112466,
-0.6422830224,
0.000115543,
0.1669116616,
-0.6285805702,
0.2326985002,
-0.0832130685,
0.0245005433,
-0.1535637528,
-0.0242395606,
0.0609050691,
-0.106129542,
0.0199470464,
-0.0505509488,
-0.2283892334,
-0.2481738329,
0.6387435198,
-0.0299810339,
0.1081740111,
-0.6304483414,
0.3527700603,
0.2514102459,
-0.2475285232,
-0.3831180334,
-0.1212913916,
0.2003689408,
-0.0978030935,
-0.2113644928,
0.4761022925,
0.2251046449,
0.399802655,
0.0064952285,
0.2575468123,
0.0505141579,
0.0444828942,
0.2104926407,
-0.1847690642,
0.0873618722,
-0.0954156145,
-0.1755374521,
0.0191609282,
-0.2041369975,
0.0782018974,
0.1050146371,
-0.2045366764,
0.1093199328,
-0.2950010598,
0.0116040986,
-0.2338980734,
-0.30991593,
0.1454840899,
0.3009775281,
0.1118348613,
-0.0831262693,
-0.1830655187,
0.2358212471,
-0.2309439033,
-0.0270279665,
0.001254426,
-0.3378607929,
-0.0525658131,
-0.1386359632,
0.5303154588,
0.1911534369,
0.0668934733,
-0.015223763,
0.122270368,
0.1313401312,
-0.0362394191,
-0.0084719677,
0.007146006,
0.4104354978,
0.2127814144,
-0.0609661341,
0.1045980975,
-0.1168992966,
0.4943226576,
0.1455180943,
-0.1304632574,
-0.3501488864,
0.1002555043,
-0.1553599238,
0.0072968714,
-0.3188312352,
-0.0977082998,
-0.2095629871,
0.1084098294,
-0.2698789239,
0.7121252418,
0.2677801847,
0.2180770785,
0.1329805851,
0.1029776633,
-0.0710623339,
-0.408136338,
-0.0947026089,
-0.0753436163,
-0.0411891192,
-0.0888822824,
0.0353819095,
-0.0306442641,
0.3885797858,
-0.1165231317,
0.1027396247,
-0.4580666423,
0.0715833232,
-0.0804569498,
0.100264065,
0.1204329282,
-0.2551064789,
0.2448671758,
-0.4125899374,
0.0325443558,
-0.1087880135,
-0.4531965256,
-0.1931726038,
0.0459405407,
0.0540225618,
0.1399485618,
0.0338589661,
-0.172211051,
-0.0154425651,
-0.0375099257,
-0.162090376,
-0.16273202,
0.056396015,
0.1628102511,
0.0724286214,
-0.3136123419,
0.1613570601,
0.1359166354,
-0.3148086667,
-0.6189990044,
0.3667177856,
0.1239369735,
-0.1368637234,
0.2416619807,
-0.119421415,
-0.0519924425,
0.5253448486,
-0.6610502601,
-0.053631451,
0.0278384853,
0.1480033547,
-0.3420060277,
0.368570596,
0.367085129,
0.0587694719,
0.0175579339,
-0.0795673281,
-0.2193675637,
0.0355436951,
0.198166728,
0.3416230381,
-0.1488902122,
0.1682679802,
-0.0125523098,
0.3555632532,
-0.0052119144,
-0.1876686066,
0.3496346772,
0.276019007,
0.5141161084,
-0.1892975867,
-0.1055217162,
-0.5744798183,
-0.1770073175,
-0.1977813691,
0.030764807,
0.1037042886,
-0.0454977378,
-0.1361049265,
-0.1728488356,
-0.3196502328,
-0.1677066386,
0.0215868186,
-0.380263716,
0.3766801357,
0.1258679777,
0.2262200117,
0.0465900302,
-0.1971981972,
-0.0069377678,
0.2207195163,
0.3263596594,
0.076213643,
-0.1963423938,
0.0560264103,
0.0341769345,
0.2427734584,
-0.1556183398,
-0.029406121,
0.1411689371,
-0.1030971929,
0.0389270522,
0.3316909373,
0.736864686,
0.0918534771,
0.0934491679,
0.072722733,
-0.3702743649,
-0.2972917855,
-0.0185838267,
0.0517492965,
-0.2108954489,
-0.1065100431,
0.6030523181,
0.1897176057,
-0.0930287912,
-0.3880623281,
0.4908542037,
-0.3521109819,
-0.4243789315,
-0.1121426076,
-0.0784951746,
-0.4928721189,
-0.1960447431,
-0.3165829778,
-0.1781642437,
-0.1199571639,
0.0184130091,
-0.3197627962,
-0.1178012341,
0.0356112197,
-0.1558903158,
0.3335535824,
0.306892097,
0.0824252814,
0.1043406278,
0.1859697551,
0.0567438044,
0.3127239645,
-0.1555327326,
-0.0530605987,
-0.1701952964,
0.202626422,
0.0648549795,
0.0702833608,
-0.0058355322,
-0.0307653379,
-0.1382613778,
-0.2153842449,
-0.2612842917,
-0.0104755033,
-0.2210034579,
-0.0227102358,
-0.1755325943,
-0.487475723,
0.4563179314,
-0.1010736674,
-0.1883664578,
-0.1476009339,
-0.0701861456,
-0.3030478954,
0.1635480672,
0.1351787001,
0.8293325305,
-0.0081276018,
0.1564805806,
0.063527517,
-0.1072185338,
0.0256157834,
-0.1322308481,
-0.0666986853,
-0.3328027427,
-0.2906269729,
-0.1825675219,
-0.1834263802,
-0.0186115876,
0.3404779136,
-0.3613781035,
0.3638463318,
-0.2523616254,
-0.0129182572,
-0.0684402287,
-0.0061907643,
-0.0827886537,
-0.239255473,
-0.1205002144,
0.0178154744,
-0.1263614744,
0.0206516385,
-0.0170569718,
0.1329818219,
0.1653864682,
0.088345021,
0.3602746725,
0.0859847665,
-0.0614528954,
0.3906389475,
-0.1673135608,
-0.4016439021,
-0.1059150621,
0.6008501649,
0.4341291189,
0.0510472544,
0.0976217613,
-0.0349938534,
0.0490862019,
0.299687624,
-0.1671099067,
-0.0298145767,
0.4338350594,
0.2377778441,
-0.00864607,
0.0113463141,
-0.1001395136,
-0.1390535533,
-0.0175677445,
-0.0650365949,
0.0446573086,
-0.3660845459,
0.0471703224,
0.2655338943,
0.3460133672,
0.0343471952,
0.0075052227,
0.0799463391,
0.0904639214,
0.3925040066,
0.0361484326,
-0.3290791512,
0.0518722571,
0.5086250901,
0.1846596003,
-0.0978843272,
0.2865215242,
-0.2758655548,
0.0242178701,
-0.1840949506,
0.413281709,
0.0370380469,
-0.2001433223,
0.1599384099,
0.0937232226,
0.438400358,
0.0149283875,
-0.1348419487,
0.0453366712,
0.0022418518,
0.0129868435,
-0.0573016033,
-0.316382587,
0.1181781814,
0.246770218,
0.3534680307,
0.3582064211,
-0.2377297729,
-0.0091209868,
0.4705206156,
-0.1857518405,
0.1302443892,
0.3519061804,
0.329446882,
0.2843286395,
0.1291948557,
-0.2915622294,
-0.3496344686,
-0.0622044019,
-0.2297858447,
-0.3173733056,
-0.1254489869,
0.0059911208,
0.1760306358,
-0.0534442104,
0.1571172774,
-0.3403256238,
-0.4503935277,
0.2765609026,
-0.2510400414,
0.1360722929,
0.1708583832,
0.0842052996,
0.1272509396,
0.1955583245,
0.4348478019,
-0.2793289125,
-0.0205026455,
-0.2099933028,
0.0822774768,
-0.1371682882,
-0.047650747,
0.0680944324,
-0.1127317324,
-0.5614902377,
0.0071767056,
0.7099935412,
0.1469893456,
0.0845733508,
-0.2261670232,
0.0306614749,
0.154983148,
0.2425204515,
0.0265948121,
-0.2630412579,
-0.3262081444,
0.322283417,
0.1072233617,
0.0007807052,
-0.1187381297,
0.2667147517,
-0.1083326489,
-0.2915619016,
0.166012913,
0.1182731017,
0.0491999276,
-0.1892534941,
0.0828058198,
0.414696306,
-0.2138019204,
0.32868132,
0.5098993182,
-0.216927126,
0.0500129014,
0.1384070367,
0.3438335359,
-0.1801336557,
0.4385667145,
0.0227652807,
0.4802251756,
-0.0042777788,
0.0963329673,
-0.0411803201,
-0.4797661602,
0.0866852626,
0.0685353205,
-0.2614328861,
0.0901099816,
0.038750235,
0.0042927065,
0.171146661,
-0.3964393735,
-0.1746974736,
0.480050981,
-0.1526479423,
0.0949189067,
0.1018083319,
-0.1112463027,
0.2824839056,
0.211182788,
-0.0595506765,
-0.2754019499,
0.4276596308,
0.1697071195,
-0.0429922529,
0.0644589216,
0.0970564187,
0.1154243052,
-0.1176580489,
-0.1695507169,
0.0883060247,
-0.1025522649,
0.0809190497,
0.0268962551,
-0.0096897408,
-0.2110144198,
0.0987924635,
0.0845461488,
0.3834222555,
0.3057736456,
-0.0233611166,
0.1660705656,
0.1643570364,
-0.157023415,
-0.0190254357,
0.1402455568,
-0.0021698833,
0.0448977984,
0.2179056108,
-0.0300383456,
0.4400351048,
-0.3797804415,
0.238194257,
0.3017784655,
-0.1042672172,
-0.1633945554,
0.0579112992,
0.1083802953,
0.0334221907,
0.5928037763,
-0.3671621382,
-0.0240954198,
0.0383852869,
0.0305531342,
0.0665904507,
0.4713378251,
0.275586307,
-0.3269462287,
-0.2479608804,
-0.149293676,
-0.5072790384,
-0.0564655513,
0.3451334834,
0.3302532732,
-0.3488495946,
0.090183571,
0.0922685862,
0.2255482674,
-0.0054459767,
-0.1682596803,
0.1758975089,
0.3226551414,
0.0473419689,
-0.2322091311,
-0.0225882921,
0.293155551,
-0.1141256765,
-0.1815204322,
0.1177122444,
0.1478694528,
-0.017084837,
0.1777738184,
0.1491096616,
-0.069270581,
0.3727111518,
0.563862443,
0.0122296941,
0.0836878046,
-0.1030978337,
0.3902325332,
0.3161498904,
-0.1495485157,
-0.0536089279,
-0.148792997,
0.068230398,
0.1575759649,
-0.507230401,
0.2509380877,
-0.1679800302,
-0.2084991783,
0.0972753838,
0.154070586,
-0.4413479269,
-0.084566094,
0.0100881355,
0.1932951212,
0.0599629469,
0.3546243608,
0.0319329016,
0.4073847532,
-0.1656412333,
-0.1417230219,
0.3911100328,
-0.1909820884,
-0.2934278548,
-0.1084173396,
0.249672398,
-0.1243686825,
-0.1343961209,
-0.3410141766,
0.1087985784,
0.4196591377,
-0.2753858566,
-0.0127334418,
0.4381883144,
-0.142344743,
0.1340464503,
0.0069053038,
0.5526455641,
0.1068051234,
-0.2677078545,
-0.0825626105,
-0.216901809
]
|
https://github.com/huggingface/datasets/issues/1993 | How to load a dataset with load_from disk and save it again after doing transformations without changing the original? | I plan to update the save_to_disk method in #2025 so I can make sure the new save_to_disk doesn't corrupt your cache files.
But from your last message it looks like save_to_disk isn't the root cause right ? | I am using the latest datasets library. In my work, I first use **load_from_disk** to load a data set that contains 3.8Gb information. Then during my training process, I update that dataset object and add new elements and save it in a different place.
When I save the dataset with **save_to_disk**, the original dataset which is already in the disk also gets updated. I do not want to update it. How to prevent from this?
| 37 | How to load a dataset with load_from disk and save it again after doing transformations without changing the original?
I am using the latest datasets library. In my work, I first use **load_from_disk** to load a data set that contains 3.8Gb information. Then during my training process, I update that dataset object and add new elements and save it in a different place.
When I save the dataset with **save_to_disk**, the original dataset which is already in the disk also gets updated. I do not want to update it. How to prevent from this?
I plan to update the save_to_disk method in #2025 so I can make sure the new save_to_disk doesn't corrupt your cache files.
But from your last message it looks like save_to_disk isn't the root cause right ? | [
-0.3105080724,
-0.0680622831,
0.0046884213,
0.2137425393,
0.3093695939,
0.090576224,
-0.0354268625,
0.0527886935,
-0.0732768625,
0.006163137,
0.0222794786,
0.3242502511,
0.0898375958,
0.1039880365,
0.1767605543,
0.2547084987,
0.2500335276,
0.1937623173,
-0.3340505362,
-0.026814444,
-0.2293638736,
-0.1129302755,
0.1087109819,
-0.2835573554,
-0.3304686546,
-0.3502231836,
-0.0617364086,
0.0674630776,
0.101074934,
0.0025960819,
0.1650635451,
0.160416007,
0.2431451231,
0.2890100181,
-0.0001189022,
0.0036651373,
-0.2793878913,
-0.0914112926,
-0.3182114661,
-0.0627908483,
0.023771381,
-0.1687757224,
0.0643621609,
-0.2784352005,
-0.026873352,
0.0422445163,
-0.1302839667,
-0.2041806132,
0.6294940114,
-0.2092859447,
0.1225879863,
-0.1459359974,
-0.1816229224,
0.026853621,
-0.2328370661,
0.2694655359,
0.0554414093,
0.039373789,
0.0392527469,
0.3304747939,
-0.089667879,
0.2254992723,
-0.3003199995,
-0.2334359288,
0.4113833606,
-0.0618236884,
0.1770337671,
-0.3527987599,
0.1796049029,
-0.0407532267,
0.8042437434,
-0.3945490122,
-0.2202159166,
-0.2704584002,
0.0771652386,
-0.1269114763,
0.18843247,
0.0927261487,
0.0741950199,
0.1356611103,
-0.5429515839,
-0.8058212399,
-0.0792826042,
0.0439731702,
0.0849198103,
-0.5327283144,
-0.0286310818,
0.1143598035,
0.269880861,
0.280354768,
0.5117333531,
-0.1818696409,
-0.2572779953,
0.1177859753,
-0.2956848443,
-0.2979615033,
-0.2101858705,
0.200738132,
-0.0370491184,
0.2396806628,
0.1317306161,
-0.0441242866,
-0.2862706184,
0.0730635002,
0.1336909086,
0.3349232972,
0.0764578283,
0.1115131825,
0.0928424746,
0.0828307718,
-0.1914320588,
-0.0887875408,
0.3011988401,
-0.0253596604,
0.6220220327,
-0.2496525496,
0.3028305173,
-0.0809739456,
0.1319756657,
0.0198020209,
0.1669227034,
-0.030759275,
-0.1410050541,
0.2388069034,
0.1260762066,
0.2384851575,
0.1625215858,
0.2277151495,
-0.0890765339,
-0.0952498019,
-0.19749403,
-0.0420267247,
-0.2991293073,
0.4488774836,
0.1797112226,
-0.0550354086,
0.0623020977,
0.4448397458,
-0.426871419,
-0.1361712664,
-0.0558105446,
-0.2085803151,
0.3360282779,
0.2015106529,
0.1050625369,
0.2018718272,
-0.0205758587,
-0.0619851425,
-0.0804888234,
0.6188936234,
-0.436011821,
-0.2547795475,
0.0720280185,
0.1330968291,
-0.1292629391,
0.1620910317,
-0.6500720382,
0.1481629163,
0.1823385805,
-0.1603375673,
0.2286166102,
0.0785052925,
-0.3790595829,
-0.2501143813,
-0.0768621042,
0.2681691051,
-0.3776040673,
-0.0919736102,
0.142182827,
-0.1908733398,
-0.0786425471,
0.2338196039,
-0.3230122328,
0.256549865,
-0.2728489041,
-0.2951152325,
0.5308727026,
0.1095688343,
-0.4193350673,
0.1607095897,
-0.0415810868,
-0.0676336884,
0.0187849849,
0.5070464015,
0.1284544021,
-0.212523371,
-0.3108477294,
0.2478175163,
0.1098065302,
0.0558845513,
-0.0957123041,
-0.1768735945,
0.2356046438,
-0.2986459136,
-0.061473798,
0.4517142773,
0.2861812711,
0.4201847911,
0.2112656385,
0.0092201587,
0.0990409851,
0.3704632521,
-0.0746404529,
-0.0521769524,
-0.2253955156,
0.206077382,
-0.6755853891,
0.0409301072,
0.1792388707,
-0.6058936119,
0.3495006859,
-0.1251086742,
-0.0100671835,
-0.2055464536,
-0.0684827566,
0.028656166,
-0.0467947684,
0.0033017516,
-0.0353207439,
-0.1637222469,
-0.2710680068,
0.533338666,
-0.084581539,
0.0758437961,
-0.6207579374,
0.3459843099,
0.1316462606,
-0.1627103686,
-0.3209211826,
-0.0708474442,
0.2944805324,
0.0222208854,
-0.1368242949,
0.3887174726,
0.1961943209,
0.4301019907,
0.0012598735,
0.2552749813,
0.0939521343,
-0.0422645323,
0.0979754776,
-0.1994078755,
0.1112074479,
-0.130440563,
-0.1259729713,
-0.0568818487,
-0.2236303687,
0.0619359314,
0.0439174101,
-0.2145468742,
0.0435214341,
-0.3417888582,
0.0263667144,
-0.2048582286,
-0.2158521861,
0.1839472204,
0.3429166973,
0.159992516,
-0.1657074541,
-0.1038521007,
0.2246286124,
-0.2585545182,
-0.0839283168,
0.0609278791,
-0.1497136652,
-0.096774511,
-0.1595598757,
0.5698389411,
0.1799430549,
0.0722896382,
0.0952889994,
0.1322449446,
0.0780078843,
-0.0757002383,
0.0063115521,
-0.009838813,
0.5663112998,
0.2622885108,
-0.035102997,
0.1173706204,
-0.151257053,
0.4364985824,
0.1587659568,
-0.0320276059,
-0.3339219391,
0.0973033458,
-0.1207988113,
0.0436149463,
-0.3793648183,
-0.1152436212,
-0.2534186542,
0.0617880523,
-0.1707231551,
0.7472001314,
0.2428153008,
0.1477638632,
0.0102006085,
0.1077872068,
-0.1298885643,
-0.3767109513,
-0.1586436331,
0.1013626903,
-0.0191386454,
-0.051520735,
0.125658825,
-0.0810724944,
0.3847854435,
-0.0449681841,
-0.0184503216,
-0.5178276896,
0.0705106705,
-0.0671276972,
0.0722048357,
0.0966843665,
-0.1959408671,
0.1818970442,
-0.3614749312,
-0.0663397312,
-0.1263328791,
-0.4590772986,
-0.180594638,
0.0563870519,
0.001703198,
0.2645660639,
-0.0602226965,
-0.2389340699,
0.0197037309,
-0.0604272299,
-0.1862341017,
-0.2104735374,
0.0663477257,
0.0624298006,
0.1308453828,
-0.2201141566,
0.2857636511,
0.0032418566,
-0.3236315846,
-0.6576063037,
0.4279029965,
0.1779449135,
-0.200141266,
0.312207222,
-0.1104268208,
-0.0269731805,
0.4068781137,
-0.5890812278,
-0.1938370913,
0.0083892886,
0.1047085151,
-0.4142181277,
0.2215415835,
0.3652361333,
-0.0205240026,
-0.0421428382,
-0.0373717621,
-0.2552670836,
0.0953871682,
0.154339388,
0.4772269428,
-0.2085596919,
0.2322047651,
0.0358265713,
0.3859618306,
-0.0511955097,
-0.0470176004,
0.3821455538,
0.2632108927,
0.5747000575,
-0.2582439482,
-0.1334561408,
-0.5062973499,
-0.2519564629,
-0.3096194565,
-0.0341154635,
-0.0407003649,
-0.0676546991,
-0.1260347366,
-0.2426860183,
-0.217879653,
-0.1950589269,
0.0797049701,
-0.288982749,
0.3272452056,
0.0038286361,
0.1908488572,
0.1868159324,
-0.2061287612,
-0.0138953887,
0.2137176096,
0.3998019099,
0.0800073743,
-0.3275632858,
0.0140289934,
-0.2869058251,
0.2075459659,
-0.2099594474,
0.0030590012,
0.0532291122,
-0.01384125,
0.0894815251,
0.2036098391,
0.7742395401,
0.1549129337,
0.1421177536,
0.1629954278,
-0.4092797935,
-0.2115703672,
0.0028168508,
0.0995187014,
-0.2312851399,
-0.1408342719,
0.6834016442,
0.1378548145,
-0.1164825857,
-0.4072920978,
0.4617177844,
-0.2899163067,
-0.2482812405,
-0.1648036093,
-0.2642419934,
-0.4946280718,
-0.149438858,
-0.2242548317,
-0.1094838977,
-0.1916746944,
0.1237000525,
-0.1940565854,
-0.1066659689,
0.0562277101,
-0.183599785,
0.3257077336,
0.3064142466,
0.0279382616,
0.1640269011,
0.1848331988,
0.1314573884,
0.3027798831,
-0.1124106124,
-0.0372947007,
-0.0790413767,
0.1409603357,
0.0719051734,
0.1694459319,
0.0061107329,
0.0176247228,
-0.011918447,
-0.1746779382,
-0.2852498889,
-0.072213158,
-0.1454938203,
-0.171391204,
-0.2936676145,
-0.4344514906,
0.5158484578,
-0.0422945097,
-0.1093989238,
-0.1100788787,
-0.1075984314,
-0.2939422727,
0.1297606379,
0.2262260169,
0.9243402481,
0.0883859843,
0.1814994514,
0.0876086801,
-0.1721742302,
0.0167081784,
-0.0423725098,
-0.0463221855,
-0.3026046157,
-0.365731895,
-0.1760590971,
-0.144395709,
0.0477700792,
0.263994813,
-0.3115509748,
0.3357238173,
-0.2909371555,
-0.1034817696,
-0.1046893224,
-0.0075613498,
-0.2122483402,
-0.2458736598,
-0.2050827891,
0.0313248411,
-0.1515482515,
-0.027383741,
-0.0318108238,
-0.0045626718,
0.2649884224,
-0.00175852,
0.3558605015,
0.1569264829,
-0.0803070441,
0.2989882529,
-0.1692278534,
-0.4347156286,
-0.0530973002,
0.5133100152,
0.4914638698,
0.0112368474,
0.0094529791,
-0.0553231239,
-0.0235152747,
0.3598998487,
-0.1810909212,
-0.1940714121,
0.486232549,
0.0869536549,
-0.0590407178,
0.0497814752,
-0.0420020223,
-0.291921705,
-0.0474306829,
-0.1287676394,
0.1325778812,
-0.2795439959,
-0.0459130034,
0.2894845605,
0.273804009,
-0.0742636845,
0.0540253259,
0.0834546238,
0.0090324096,
0.3221890926,
-0.0452657565,
-0.3151635826,
0.0543161295,
0.5431887507,
0.1804058254,
-0.0464719944,
0.3360171318,
-0.3092155755,
0.0523006059,
-0.2045598924,
0.3989335895,
0.1732901782,
-0.1432103813,
0.1380077749,
0.020450687,
0.443957448,
-0.0311350115,
-0.024284875,
0.0992706195,
-0.0084160585,
0.1196402535,
-0.0480962321,
-0.3914591968,
0.1300691515,
0.2458520532,
0.3818081915,
0.32046628,
-0.1763055325,
0.0028131944,
0.3804043531,
-0.2188311368,
0.1583357006,
0.2809141874,
0.2071996033,
0.2206033319,
0.1162377372,
-0.2657071352,
-0.328017652,
-0.051455304,
-0.1594759077,
-0.3051246405,
-0.1652149111,
-0.0302932765,
0.136443615,
-0.0375770181,
0.189955011,
-0.2565505505,
-0.4506434798,
0.2677791715,
-0.1295458674,
0.1429376304,
0.2473305762,
0.205123201,
0.1521452367,
0.0653467178,
0.4487792253,
-0.2328965664,
-0.1185056791,
-0.2105094045,
0.1233187839,
-0.0207087975,
-0.0022416855,
0.0580325238,
-0.1482615471,
-0.5246191025,
-0.0672188699,
0.5569462776,
0.1424942315,
-0.076521188,
-0.2013105005,
0.0068911882,
0.2139703631,
0.2115524411,
0.0510492474,
-0.34327057,
-0.2787158489,
0.3432044983,
0.1442660242,
-0.016044436,
-0.2254811674,
0.2461639047,
-0.1806958765,
-0.3061956763,
0.1442253143,
0.1767638028,
-0.0585686862,
-0.2754855752,
0.158903569,
0.2719601393,
-0.3255972862,
0.3828685284,
0.5380004644,
-0.2591250539,
0.009626166,
0.2257220745,
0.2819714844,
-0.1335762143,
0.4269306958,
0.0098502301,
0.5303926468,
-0.0395825244,
0.1332314461,
-0.0633736625,
-0.4151785374,
0.0702957809,
0.1649890691,
-0.2766587734,
0.060055811,
0.0243206285,
-0.0979801714,
0.1432590932,
-0.4174813032,
-0.1507121474,
0.4192140996,
-0.2484191507,
0.1074254438,
0.0579754487,
-0.1120398566,
0.2826023102,
0.2744212151,
-0.0536197163,
-0.2268413603,
0.4427160323,
0.1255391091,
-0.1913320422,
0.2044382691,
0.1249770597,
0.1588973403,
-0.0993125662,
-0.1169832647,
0.1147719175,
-0.0848858654,
-0.0037678101,
0.0291743018,
0.029879,
-0.1809428483,
0.1118037254,
0.1676975042,
0.2783719599,
0.3059470057,
-0.0228865631,
0.1679927707,
0.0827449188,
-0.0256153066,
-0.0337704197,
0.212232843,
0.0137036322,
0.0140267713,
0.3118377626,
-0.0362617113,
0.4630797505,
-0.3419649601,
0.2062889934,
0.4129407108,
-0.1074001491,
-0.1485199332,
0.0904574394,
0.1278406978,
-0.0378110074,
0.5705618262,
-0.2957134843,
-0.0160772186,
0.0124919638,
0.0395254716,
0.1230499744,
0.4332266748,
0.2128033191,
-0.4697757959,
-0.2823476791,
-0.1664896011,
-0.6273466945,
-0.0508996062,
0.3253655732,
0.3701801598,
-0.225856483,
0.1118431687,
0.0707367063,
0.2098542899,
0.0369165652,
-0.2351110727,
0.1503261775,
0.2999606729,
0.1234921142,
-0.1595017314,
0.0503460541,
0.2770723701,
-0.1349765956,
-0.2388399243,
0.1724777818,
0.1970548332,
-0.0143193435,
0.2137269825,
0.1389215291,
-0.1556821764,
0.4832549989,
0.5390318036,
0.1382324547,
0.0534415878,
-0.1822286397,
0.3323270082,
0.2797114551,
-0.0581520312,
-0.1694721878,
-0.1795462966,
0.0746412352,
0.1931519657,
-0.3903321326,
0.3852841258,
-0.2698163986,
-0.0080450559,
0.0730059221,
0.1210963055,
-0.5197297931,
0.0209047049,
0.1311254799,
0.1718315482,
0.1720627248,
0.4176316559,
0.0450439118,
0.3535894752,
-0.2674762011,
-0.1531488448,
0.4640334547,
-0.1586668938,
-0.3031256497,
-0.1587206721,
0.3097461164,
0.0269457903,
-0.0641722083,
-0.3660286963,
0.0210535917,
0.3587174416,
-0.2671586573,
-0.0269524362,
0.5555732846,
-0.2037760913,
0.1576040685,
0.0878341123,
0.5945923924,
0.0969751701,
-0.3053184748,
-0.0882794261,
-0.3290878832
]
|
https://github.com/huggingface/datasets/issues/1993 | How to load a dataset with load_from disk and save it again after doing transformations without changing the original? | ok, one more thing. When we use save_to_disk there are two files other than .arrow. dataset_info.json and state.json. Sometimes most of the fields in the dataset_infor.json are null, especially when saving dataset objects. Anyways I think load_from_disk uses the arrow files mentioned in state.json right? | I am using the latest datasets library. In my work, I first use **load_from_disk** to load a data set that contains 3.8Gb information. Then during my training process, I update that dataset object and add new elements and save it in a different place.
When I save the dataset with **save_to_disk**, the original dataset which is already in the disk also gets updated. I do not want to update it. How to prevent from this?
| 45 | How to load a dataset with load_from disk and save it again after doing transformations without changing the original?
I am using the latest datasets library. In my work, I first use **load_from_disk** to load a data set that contains 3.8Gb information. Then during my training process, I update that dataset object and add new elements and save it in a different place.
When I save the dataset with **save_to_disk**, the original dataset which is already in the disk also gets updated. I do not want to update it. How to prevent from this?
ok, one more thing. When we use save_to_disk there are two files other than .arrow. dataset_info.json and state.json. Sometimes most of the fields in the dataset_infor.json are null, especially when saving dataset objects. Anyways I think load_from_disk uses the arrow files mentioned in state.json right? | [
-0.3007223308,
-0.0692068338,
-0.0054889163,
0.2683241069,
0.1868758351,
0.0893004835,
-0.09074191,
-0.0502164736,
-0.0936115384,
-0.0296892803,
0.0845306218,
0.3922943771,
0.0921067819,
0.2010049224,
0.1915562898,
0.2176254541,
0.2755787075,
0.2541548014,
-0.2981148362,
-0.0849449262,
-0.1826586425,
-0.2011494637,
0.0351460651,
-0.2450300902,
-0.2057039291,
-0.3581504524,
-0.0677712485,
0.0153926043,
0.1225501001,
0.0245492421,
0.1340075433,
0.0892244279,
0.2528146207,
0.2696576715,
-0.000121676,
0.0475671887,
-0.3047076762,
-0.1001052856,
-0.3526190519,
-0.1412884295,
-0.0697200447,
-0.2212414891,
0.0719518661,
-0.3070665002,
0.0589957237,
-0.2824420035,
-0.1260731667,
-0.2450783551,
0.6672536731,
-0.2722017169,
0.0699747056,
-0.1284600496,
-0.1617944688,
0.0976970121,
-0.2888135314,
0.3928674459,
0.1804586202,
0.0834340677,
-0.0248981025,
0.3822443485,
-0.0219695941,
0.1612482369,
-0.200020358,
-0.2527585328,
0.3833416104,
-0.0076436126,
0.2212194055,
-0.2953318655,
0.1467777342,
0.0135786366,
0.8064633012,
-0.405023098,
-0.2574878037,
-0.2882361412,
0.1536289155,
-0.0580589883,
0.1496301889,
0.2156997919,
0.0218877904,
0.1402098686,
-0.5136812329,
-0.868442595,
-0.126684323,
0.1264939159,
0.1287237108,
-0.5532696247,
-0.0229648184,
0.1637459695,
0.2024899423,
0.2185243517,
0.4860470593,
-0.3468030095,
-0.2665129304,
0.1081001684,
-0.2416038811,
-0.257950455,
-0.3543599248,
0.1011799499,
-0.0114109498,
0.2894116044,
0.1406662315,
-0.0836776122,
-0.3567639291,
0.1603851318,
0.2414060533,
0.2809043527,
0.2183112651,
0.169306621,
0.0739972293,
-0.0631904751,
-0.1861830205,
-0.0970508382,
0.186216414,
0.0415193215,
0.5600596666,
-0.3111511767,
0.2295673043,
-0.012257372,
0.150287196,
0.0228206031,
0.0895474702,
-0.1179457083,
-0.1425841451,
0.2670942545,
0.1297620982,
0.2194280326,
0.1593542993,
0.2407313883,
0.0423475616,
-0.037606135,
-0.1410648674,
-0.0918836147,
-0.2097061723,
0.4661526978,
0.0937510803,
-0.0388413221,
0.1977844536,
0.4313408136,
-0.4969686568,
-0.1085933149,
0.0501843914,
-0.1930904984,
0.3274523914,
0.1736688018,
0.1454109401,
0.2489248067,
-0.0879917741,
-0.0936398506,
-0.1191325635,
0.5767198801,
-0.3575343192,
-0.1520614028,
0.0701174662,
0.1043709144,
-0.159828648,
0.0983542725,
-0.8150242567,
0.1427524686,
0.0247535594,
-0.1231688336,
0.1728977114,
0.0534342267,
-0.2563693821,
-0.2302291244,
-0.0512652434,
0.1930935532,
-0.4120172858,
-0.1680331677,
0.1258453131,
-0.2549982369,
-0.1799415648,
0.2108703256,
-0.3636851907,
0.2950892448,
-0.2587228715,
-0.3023215234,
0.6723538637,
0.0204601437,
-0.3387462795,
0.1760645211,
-0.0282903183,
-0.1184443086,
0.0147041483,
0.5250899196,
0.1372102797,
-0.0953127667,
-0.4145095646,
0.2945403755,
0.1407809108,
0.0868425891,
0.0795525685,
-0.2047656476,
0.1286369413,
-0.2869850695,
-0.2456306666,
0.3859401941,
0.2542549074,
0.3583353758,
0.3105890155,
0.0150289983,
0.1410469413,
0.4581475854,
-0.1164279431,
0.0385381468,
-0.2127674818,
0.178436771,
-0.7484531999,
-0.00657159,
0.2338046134,
-0.6842120886,
0.3345312178,
-0.1203259975,
-0.0277767964,
-0.1687025428,
-0.0664195493,
0.1298466474,
-0.0762264282,
0.0424032025,
-0.0529154502,
-0.1547597945,
-0.2675636113,
0.3820065856,
-0.0523814783,
0.1227223128,
-0.6055696607,
0.3676996827,
0.2111805826,
-0.200248301,
-0.2397425175,
-0.193133831,
0.2055211067,
0.0092982994,
-0.0893528759,
0.3786781728,
0.1809557825,
0.4551724494,
0.0918439403,
0.2322871536,
0.0534292236,
-0.0460144095,
0.1447262764,
-0.1604726911,
0.1452555805,
-0.1221863031,
-0.2638005316,
0.0442184657,
-0.2015414238,
0.1787592322,
0.1345493793,
-0.1926781237,
0.0233342368,
-0.2902032435,
-0.0180923939,
-0.222067371,
-0.2539128959,
0.1853526384,
0.3977226913,
0.126713708,
-0.1721723676,
-0.1254345626,
0.2959312201,
-0.2575978041,
-0.0214609969,
-0.0102390442,
-0.3651689589,
0.0082923295,
-0.094108969,
0.6046717763,
0.161114186,
0.0820365325,
0.0866395831,
0.0911178812,
0.1511916816,
-0.0454357043,
0.044233337,
-0.010149396,
0.5799142718,
0.2443164438,
-0.0383159593,
-0.0365615599,
-0.1149246246,
0.3536055982,
0.1652427018,
-0.0946869627,
-0.2773091495,
0.1180242747,
-0.2011265904,
0.0713498965,
-0.488460809,
-0.1273128688,
-0.2521908879,
0.1512747258,
-0.271494329,
0.7723133564,
0.2325680405,
0.1263020635,
0.1219077781,
0.0154314013,
-0.1470240355,
-0.43889606,
-0.0966936871,
-0.0195643082,
-0.0103023732,
-0.0622431934,
0.1154476702,
0.0493931361,
0.3781048357,
-0.0810579285,
-0.0131593384,
-0.4396047592,
0.1891237646,
-0.0656803325,
0.1645983309,
0.0659914389,
-0.221565336,
0.2795462608,
-0.3811887205,
-0.0384198166,
-0.0594332032,
-0.3607290089,
-0.2850465178,
-0.0065298127,
0.0134895509,
0.2896595001,
0.0172298402,
-0.1902122051,
0.0335530341,
-0.0402981266,
0.0173521172,
-0.1225783229,
0.0557491221,
-0.0140361646,
0.2444536686,
-0.1130923256,
0.1804328561,
0.0764265731,
-0.211416766,
-0.5961000323,
0.4128050208,
0.1633278877,
-0.1741424501,
0.3108078241,
-0.1741732359,
-0.0210466832,
0.3101856112,
-0.6087192297,
-0.1141922474,
0.0600614063,
0.1037477031,
-0.3919461071,
0.3361912966,
0.3062701225,
-0.0278479252,
0.0144451186,
-0.0334716178,
-0.2389529347,
0.0784411505,
0.143777281,
0.4244505465,
-0.1612541676,
0.2261485904,
-0.0966197178,
0.3579065502,
0.0317313373,
-0.1503337175,
0.2577559054,
0.1534894258,
0.4387214482,
-0.1750788987,
-0.0522777811,
-0.5761281848,
-0.161819905,
-0.221773535,
-0.1148252562,
-0.0057710372,
0.032396052,
-0.1075937301,
-0.249658674,
-0.2805708647,
-0.1933778524,
0.0845355392,
-0.2493491471,
0.2655930817,
0.0192374233,
0.1759129614,
0.1493868679,
-0.133104682,
-0.0371736027,
0.2424815893,
0.3567497432,
0.0340545252,
-0.4226414859,
-0.0830406323,
-0.0818447545,
0.1717509627,
-0.2144636065,
-0.0884094611,
0.1600839049,
0.0011427664,
0.0677129477,
0.1965410411,
0.7361792326,
0.1874347776,
0.063700296,
0.0926947445,
-0.3455913961,
-0.3881475627,
0.084328033,
0.0506141819,
-0.2877060771,
-0.1174877882,
0.7245601416,
0.0803991929,
-0.1206823736,
-0.3576759696,
0.5562205315,
-0.3704289198,
-0.2955067754,
-0.1457946151,
-0.1664605141,
-0.5476549864,
-0.213350758,
-0.3082759678,
-0.1582364142,
-0.1307762414,
0.0646410808,
-0.2534778714,
-0.0915705487,
0.0220780205,
-0.1182215586,
0.3918709755,
0.2666741312,
0.0193667393,
0.1644687504,
0.2219797671,
0.3284146488,
0.3666436374,
-0.0153753618,
-0.0974275321,
-0.1326036155,
0.0867798254,
0.1648631245,
0.0936441198,
0.0686152652,
0.0479843765,
0.026837023,
-0.2086211145,
-0.3092782795,
-0.0560038686,
-0.1340935975,
-0.1367201805,
-0.1440846175,
-0.5415617824,
0.5702608228,
-0.0650222227,
-0.1408147067,
-0.0851876438,
-0.0459548645,
-0.2711400092,
0.1703904718,
0.1498787403,
0.8716297746,
0.1089725122,
0.1778829992,
0.1109231189,
-0.2702061832,
0.0874601752,
0.0335817821,
-0.1201459095,
-0.3685468435,
-0.1829182357,
-0.1566402614,
-0.2075344175,
0.0405542478,
0.4153391421,
-0.2801255882,
0.3261987567,
-0.2238126993,
-0.1505964398,
-0.1990750432,
0.0961726382,
-0.1657648981,
-0.1743449867,
-0.1681720763,
-0.0114011122,
-0.088193655,
-0.0306612626,
0.0053101024,
0.0413890332,
0.0553507172,
-0.0187188387,
0.3721585274,
0.084623985,
-0.0624594279,
0.2771231532,
-0.1577948928,
-0.4986291826,
-0.0826175436,
0.5926849842,
0.3960545957,
-0.0334479734,
0.0526532829,
-0.0723133832,
0.0268861204,
0.3063698113,
-0.2422437519,
-0.1719272733,
0.4481706023,
0.1376744807,
-0.0855186358,
0.1409665197,
-0.0945119485,
-0.1698207259,
-0.0376818143,
-0.0860803872,
0.0551038794,
-0.2550104856,
0.0474662594,
0.2712354362,
0.2530314624,
-0.021011468,
0.0095765805,
0.1501527876,
0.0579039045,
0.2980201542,
0.0394542441,
-0.2374411523,
0.045956187,
0.5188025832,
0.2067333609,
0.021413099,
0.4381724596,
-0.3275932372,
0.1210738868,
-0.2402664572,
0.5366312265,
0.2017429173,
-0.1746362299,
0.1869062036,
0.0694174618,
0.4007886648,
-0.047345534,
-0.02130224,
0.0853853524,
0.011167936,
0.144522205,
-0.0958095938,
-0.4380566478,
0.2343147397,
0.2624765635,
0.3535430729,
0.2583893836,
-0.2324334979,
-0.0680491701,
0.3585877419,
-0.1744464487,
0.2054148465,
0.3005223274,
0.2823811769,
0.1684187055,
0.2044437677,
-0.2087185681,
-0.3440041542,
-0.0438149795,
-0.2555530667,
-0.2365292162,
-0.1251324266,
-0.0598073341,
0.1539492607,
-0.0436909646,
0.1677891016,
-0.3266103864,
-0.4847744703,
0.2891401947,
-0.1375053227,
0.0824395493,
0.0819533095,
0.2197142094,
0.1840927601,
0.093729496,
0.448150754,
-0.3184416592,
-0.1187558025,
-0.1921022385,
0.1783019751,
-0.03281096,
0.0121086184,
0.0642224997,
-0.1316831112,
-0.6189085245,
-0.0990726948,
0.5383810997,
0.1635980904,
-0.0226290878,
-0.167563051,
-0.0290072784,
0.107552357,
0.2544226348,
0.2053808421,
-0.3422958255,
-0.2245645821,
0.3293196261,
0.1255977899,
0.0269109607,
-0.1925329417,
0.2880924344,
-0.1918520182,
-0.2873286307,
0.1465843469,
0.0319877975,
-0.0512791537,
-0.4122621715,
0.0254710373,
0.1751538813,
-0.1580881923,
0.3159532845,
0.5974051952,
-0.2331490666,
-0.0708442107,
0.14665775,
0.3994493484,
-0.0056822528,
0.3305530846,
-0.0007101989,
0.3764373362,
0.0240039285,
0.0916100144,
-0.0240888949,
-0.3671690822,
0.0081162862,
0.1389636248,
-0.2195096463,
0.0497018956,
0.0751757547,
-0.0880867168,
0.2082584649,
-0.3309558034,
-0.0835979432,
0.3510947227,
-0.1629565358,
0.082633391,
-0.1298547834,
-0.1498636752,
0.3026363254,
0.2705849409,
-0.078876324,
-0.3361344337,
0.3281603754,
0.1423033327,
-0.097157523,
0.1063297316,
0.1872469485,
0.1876195967,
-0.1655016989,
-0.1503583342,
0.0128681706,
-0.0289311223,
0.0125154685,
0.0165959988,
0.0012617977,
-0.2274464071,
0.041940216,
0.1657589078,
0.316542536,
0.3196214437,
-0.0838942155,
0.1499596685,
0.0178739037,
-0.0008009501,
-0.0735294819,
0.2127769291,
0.0151791815,
0.0294535961,
0.2632459104,
0.0044016759,
0.3795757294,
-0.3571352661,
0.2479710132,
0.3106712699,
-0.1404625475,
-0.1665807068,
0.0103726322,
0.2103222013,
-0.1065976098,
0.543431282,
-0.2923467755,
-0.0012198823,
0.0241243802,
0.0142698735,
-0.0414994806,
0.4267640114,
0.2331003398,
-0.4311104417,
-0.1866674125,
-0.1895918101,
-0.5319389701,
-0.0989798382,
0.2813745141,
0.2668111026,
-0.2489683479,
0.1820268929,
0.1321468651,
0.2249126732,
0.0598295815,
-0.1167491451,
0.1265685856,
0.3670852184,
0.1188411489,
-0.2378844768,
0.0502786189,
0.3357857764,
-0.0974641889,
-0.214331314,
0.1917598397,
0.132594347,
-0.0512678809,
0.2214892507,
0.189693585,
-0.1321160942,
0.4478757083,
0.6462354064,
0.0189493392,
0.0312895179,
-0.1774076372,
0.236267224,
0.3744186759,
-0.0216822345,
-0.1565991491,
-0.0167003013,
0.0922045037,
0.2499128431,
-0.4566820562,
0.4448709786,
-0.20418863,
-0.1868146807,
0.0137962596,
-0.0477890149,
-0.4581757486,
0.0463742875,
0.0651583821,
0.1430877298,
0.1621833146,
0.3478299379,
-0.0187163185,
0.318493247,
-0.1899689883,
-0.024314383,
0.4145452678,
-0.0772264451,
-0.1907039583,
-0.0427963734,
0.2818669379,
0.0554948151,
0.0050823055,
-0.4016093314,
0.1224806532,
0.4839179218,
-0.2663433254,
-0.0787809789,
0.3923039138,
-0.1582095474,
0.1256156117,
0.0675275996,
0.6033189893,
0.0422038436,
-0.3597432673,
-0.0921214744,
-0.2827444971
]
|
https://github.com/huggingface/datasets/issues/1993 | How to load a dataset with load_from disk and save it again after doing transformations without changing the original? | Perfect. For now, I am loading the dataset from CSV in my interactive process and will wait until you make the PR! | I am using the latest datasets library. In my work, I first use **load_from_disk** to load a data set that contains 3.8Gb information. Then during my training process, I update that dataset object and add new elements and save it in a different place.
When I save the dataset with **save_to_disk**, the original dataset which is already in the disk also gets updated. I do not want to update it. How to prevent from this?
| 22 | How to load a dataset with load_from disk and save it again after doing transformations without changing the original?
I am using the latest datasets library. In my work, I first use **load_from_disk** to load a data set that contains 3.8Gb information. Then during my training process, I update that dataset object and add new elements and save it in a different place.
When I save the dataset with **save_to_disk**, the original dataset which is already in the disk also gets updated. I do not want to update it. How to prevent from this?
Perfect. For now, I am loading the dataset from CSV in my interactive process and will wait until you make the PR! | [
-0.343549639,
-0.1861004382,
-0.0237766225,
0.1979098618,
0.2351960093,
0.0903731436,
-0.0758544207,
-0.0891272575,
-0.0024477071,
0.0613525584,
0.0818682462,
0.2888205051,
0.0089174258,
0.2742180228,
0.2110170126,
0.2311642468,
0.2571014166,
0.3034490347,
-0.3161802888,
-0.0310902242,
-0.1955247521,
-0.1905542761,
0.015779404,
-0.2773292363,
-0.1335465759,
-0.3582565188,
-0.0840452239,
-0.0099305175,
0.0723503232,
0.0670776069,
0.1237910911,
0.1421200633,
0.319794625,
0.307305932,
-0.0001188234,
0.011263527,
-0.3935618699,
-0.1174852476,
-0.2952137291,
-0.1752288491,
0.0198727641,
-0.1928230524,
0.0426132269,
-0.2823274434,
-0.0355924666,
-0.078924492,
-0.1382195652,
-0.1842430085,
0.6355668902,
-0.2757764757,
0.0882280767,
-0.1013197079,
-0.2604755461,
0.1003406569,
-0.3609257638,
0.3109509647,
0.1697656959,
0.032725621,
-0.02301188,
0.3523843884,
-0.0059228987,
0.1834036708,
-0.2202824801,
-0.2364937365,
0.368504256,
-0.0557334535,
0.1962570697,
-0.3266137838,
0.1366037279,
-0.05041866,
0.7573292851,
-0.3973723352,
-0.2224437296,
-0.2200313359,
0.2223116457,
-0.1780084372,
0.1028200164,
0.2710523307,
-0.000419326,
0.1309107691,
-0.591570735,
-0.8006386161,
-0.1265456527,
0.0599237382,
0.0174781922,
-0.600536108,
-0.0098795025,
0.1061761156,
0.2177744806,
0.2815048397,
0.4598247707,
-0.2353614718,
-0.216178447,
0.1422931254,
-0.294993639,
-0.2745670974,
-0.2943740189,
0.1907630563,
-0.0581795722,
0.1961970031,
0.0701112524,
-0.0923656598,
-0.2840209901,
0.1482523829,
0.1528834254,
0.3160925806,
0.1148206964,
0.1808681786,
0.0832407922,
0.0590581521,
-0.2331293076,
-0.0536454506,
0.1533099264,
0.0603942089,
0.5767163634,
-0.227349788,
0.1721359938,
0.0239442419,
0.0714495406,
0.0731746256,
0.1662716419,
-0.0902283341,
-0.1174423471,
0.3433510363,
0.1202498525,
0.2047811002,
0.189990893,
0.2010245323,
-0.0489034876,
-0.114174962,
-0.1551880389,
-0.0595463291,
-0.3120179474,
0.4196889102,
0.1253361851,
0.0080646416,
0.1199117452,
0.5349017978,
-0.4715518951,
-0.1450415105,
0.0144294174,
-0.1974766999,
0.3453063667,
0.1837602258,
0.1647830456,
0.1672115773,
-0.0709508732,
-0.0298971143,
-0.1003753245,
0.649728477,
-0.3161401153,
-0.109240137,
0.1016732976,
0.1262909174,
-0.1379486471,
0.1107459664,
-0.7127207518,
0.1979916245,
0.0198916011,
-0.1377771944,
0.1604964584,
0.0238326602,
-0.3551613986,
-0.1828228235,
-0.0532663539,
0.1804093719,
-0.3858018517,
-0.1600942314,
0.1927725375,
-0.2199114412,
-0.1124549732,
0.224484697,
-0.3141230345,
0.2418792695,
-0.2732776701,
-0.3577341139,
0.5291256905,
0.1001323089,
-0.33412233,
0.1677853465,
-0.0348187089,
-0.0790614188,
0.0231739581,
0.5614812374,
0.1681260914,
-0.1092801243,
-0.4543693066,
0.3388877809,
0.0994200483,
0.1335134208,
0.0695957467,
-0.1695291102,
0.2710562944,
-0.3116509318,
-0.1427362561,
0.4807794392,
0.246056661,
0.3417785764,
0.3239541948,
-0.0245122481,
0.0995800123,
0.407705605,
-0.151208207,
0.0790726393,
-0.2095584273,
0.1262253672,
-0.6138049364,
-0.0311101191,
0.1936891079,
-0.5951256156,
0.3615723848,
-0.1226513013,
-0.0642849505,
-0.2044181228,
-0.0127759818,
0.0749249086,
-0.0688731968,
-0.0022938543,
-0.1109884381,
-0.1776317358,
-0.2606809437,
0.4837685525,
-0.0590836555,
0.0931874812,
-0.6138259172,
0.3396215439,
0.1712860465,
-0.2045489252,
-0.2430604547,
-0.0967050046,
0.2103917897,
0.0319461264,
-0.0962718278,
0.3377919793,
0.1939780116,
0.4088327289,
0.0129820686,
0.199359268,
0.0663036481,
-0.0132701993,
0.0733957738,
-0.2320130467,
0.1360416561,
-0.0811843127,
-0.1756191403,
0.0617034324,
-0.2363675535,
0.089263998,
0.1581324041,
-0.2045087516,
0.0544074215,
-0.3416888714,
0.0190342031,
-0.2046748102,
-0.185868293,
0.2192385644,
0.3088556826,
0.1363541186,
-0.2268323302,
-0.2074434906,
0.2330196351,
-0.2737059891,
-0.0912177414,
0.03533585,
-0.2595541477,
0.0168844014,
-0.1204079315,
0.5437601805,
0.118866317,
0.1275319308,
0.0053327638,
0.1194350868,
0.1251199096,
0.0129796509,
0.0339160264,
-0.0343920626,
0.5523138642,
0.2647173703,
-0.0509435833,
0.0724441931,
-0.1624069512,
0.4198662341,
0.1504423767,
-0.1196848527,
-0.3011408448,
0.0994732454,
-0.1615888029,
0.0660835579,
-0.468075484,
-0.0841381326,
-0.2472437322,
0.2366381586,
-0.2964736521,
0.7885242701,
0.2594656348,
0.1625134945,
0.1108180806,
0.0459687635,
-0.1312013865,
-0.4180257022,
-0.0573596805,
0.0374373719,
0.0125369951,
-0.0483345278,
0.0752370507,
-0.0037025106,
0.4305585325,
-0.0291060992,
-0.0665600002,
-0.426974982,
0.1278461963,
-0.0823733136,
0.1029743105,
0.1104521602,
-0.2120501399,
0.2974176705,
-0.4168387949,
-0.0541328117,
-0.1244079843,
-0.4201670885,
-0.2594536543,
0.0294524785,
0.0546954535,
0.2615023553,
0.0107819168,
-0.2051880509,
-0.0094757145,
-0.0083107669,
-0.1173739359,
-0.1713139415,
0.0468002632,
0.0203506704,
0.1569826752,
-0.2946604192,
0.2539894879,
0.0822967216,
-0.2376687825,
-0.5970131159,
0.3417879343,
0.159900561,
-0.1892437786,
0.289732784,
-0.1801794618,
-0.0554160327,
0.3803257048,
-0.5858958364,
-0.0945967585,
0.0443137288,
0.1413553655,
-0.4069653749,
0.3347257972,
0.3081909716,
-0.0225071013,
-0.0081291748,
-0.0677945986,
-0.2454160899,
0.0576779731,
0.1356204152,
0.3997929394,
-0.2072360069,
0.2203688622,
-0.0573366508,
0.3448047638,
-0.0246948134,
-0.1513892263,
0.289745599,
0.1356436908,
0.4245442748,
-0.1676987261,
-0.1270467639,
-0.5524553657,
-0.1735003144,
-0.2354566008,
-0.0060490468,
-0.0379286855,
0.0072154901,
-0.1281891614,
-0.2558844984,
-0.2843936682,
-0.130805999,
0.0504184663,
-0.2452313155,
0.3822574914,
0.0587057732,
0.1576601118,
0.1527378112,
-0.1986498684,
-0.0689637586,
0.2094496638,
0.3548836112,
0.0272180326,
-0.2600477338,
-0.0348768644,
-0.1532876045,
0.1102512628,
-0.2091602087,
-0.0276741311,
0.1221667081,
-0.0030596519,
0.0609428622,
0.2545266151,
0.8182936907,
0.215658024,
0.137448132,
0.0889693797,
-0.4251458347,
-0.2478493303,
0.0456908345,
0.0322487354,
-0.3078767657,
-0.0940759555,
0.6723988652,
0.1526484489,
-0.0902086794,
-0.3045026362,
0.518800199,
-0.3563798666,
-0.373773545,
-0.2266184986,
-0.1663167328,
-0.5012158155,
-0.1288880557,
-0.2598290443,
-0.0439995602,
-0.1832843423,
0.1061318517,
-0.2196142823,
-0.0676039457,
0.1095384508,
-0.1310003251,
0.3300732076,
0.3421657979,
0.0496451668,
0.1242487505,
0.2176741362,
0.1669382006,
0.3173026443,
-0.105336979,
-0.1126385927,
-0.1280664504,
0.1298923492,
0.1712063402,
0.0756070241,
0.0527423993,
0.0732394755,
-0.0068588075,
-0.1699391454,
-0.3829434216,
-0.0453670658,
-0.1490217596,
-0.0730893239,
-0.2039039284,
-0.4751251638,
0.4746287763,
-0.0925760493,
-0.1258590072,
-0.1472211033,
-0.1061395481,
-0.2921001315,
0.1399551779,
0.1306456476,
0.8632392883,
0.0326739736,
0.1525592655,
0.1198751256,
-0.170532003,
0.0168490075,
-0.0012272934,
-0.0839211419,
-0.4019956589,
-0.3279488981,
-0.1733229905,
-0.1934763342,
0.037824735,
0.325315088,
-0.32833004,
0.350751102,
-0.2487779409,
-0.1089890227,
-0.1078146473,
0.0443412997,
-0.1169935688,
-0.164963454,
-0.1856901646,
-0.0061430042,
-0.0798518956,
-0.0044730864,
-0.0543573201,
0.074077256,
0.2115780115,
0.0019405487,
0.3221925199,
0.1227276251,
-0.0707130283,
0.2805040777,
-0.1962253302,
-0.464951843,
-0.0051141325,
0.6101845503,
0.4340723157,
0.0248925686,
0.0813787803,
-0.0706976876,
-0.0266581494,
0.331471175,
-0.2104786932,
-0.1034400091,
0.4504614472,
0.136667043,
-0.1030479819,
0.0925332233,
-0.0474377051,
-0.1213084087,
0.0198433194,
-0.0646403357,
0.1135451272,
-0.350995183,
0.0355066285,
0.2403180152,
0.3644149899,
-0.0586993657,
0.031469129,
0.0625164211,
0.0130531238,
0.3171581626,
0.0708596334,
-0.2808797359,
0.0376205407,
0.5294992924,
0.2024805248,
-0.0426781327,
0.36998716,
-0.2707160711,
0.0899938419,
-0.267544508,
0.4640223682,
0.1178860441,
-0.1746008843,
0.1856896579,
0.1257214695,
0.4507122636,
-0.0159636308,
-0.0439139381,
0.0883902311,
-0.062979117,
0.1653647423,
-0.0940571129,
-0.3822159767,
0.1210727915,
0.2372950613,
0.3630729318,
0.3535116315,
-0.1228339225,
-0.0329010375,
0.3971118927,
-0.2104937285,
0.159949705,
0.280469656,
0.2849235833,
0.2143123299,
0.1544957906,
-0.3230305314,
-0.359931767,
-0.0096666915,
-0.2506663203,
-0.2394522429,
-0.1587038934,
-0.0525225438,
0.143928349,
-0.1139952689,
0.2321474403,
-0.285438329,
-0.4382479191,
0.2356993854,
-0.1452858895,
0.0925416499,
0.1704604328,
0.2144284695,
0.2009412348,
0.078536056,
0.4266559482,
-0.3415494263,
-0.1125034317,
-0.2172949016,
0.1889525354,
-0.0322937369,
0.0051216129,
0.0702453181,
-0.1169626638,
-0.6251554489,
-0.0948789045,
0.6250930429,
0.1212150455,
-0.0073650265,
-0.1670635939,
0.0100226887,
0.1534458101,
0.2904002666,
0.0841549858,
-0.3773590028,
-0.2461010069,
0.3773590028,
0.1504217237,
-0.0956228971,
-0.1158767045,
0.2852274477,
-0.2092486024,
-0.2813020349,
0.1848058254,
0.1058103293,
-0.0454827547,
-0.3390637338,
0.0373211093,
0.2553979456,
-0.1684484929,
0.3619690835,
0.5913914442,
-0.2182154506,
-0.0562766902,
0.1396618783,
0.4150112569,
-0.1396206468,
0.3794618845,
-0.0036848085,
0.3818938434,
-0.0570318848,
0.1477709264,
-0.1090394631,
-0.4267395139,
0.101137884,
0.1654105186,
-0.2610384822,
0.0225982014,
-0.0011139518,
-0.103032358,
0.1897848547,
-0.3766217232,
-0.1189317554,
0.4679529667,
-0.2189383805,
0.1519278884,
-0.0048866631,
-0.1219154298,
0.2515875697,
0.3047004044,
-0.0060797604,
-0.2621268034,
0.3338617086,
0.1696129143,
-0.1097292379,
0.0838390887,
0.0973994955,
0.2091325223,
-0.1747377664,
-0.1729256809,
0.0424712971,
-0.0594644472,
0.083061263,
0.0510299839,
0.0068145772,
-0.2014681101,
0.0683357492,
0.1713879853,
0.292863071,
0.2508703768,
-0.025109034,
0.1290168911,
0.0307843238,
-0.0182990748,
0.0967059433,
0.1933041364,
0.0061854441,
0.0254682191,
0.2639409602,
0.0461052395,
0.3334487081,
-0.3797312379,
0.2068741769,
0.4354175627,
-0.090281941,
-0.2419116199,
0.0280012581,
0.212584272,
0.0150208324,
0.5192962885,
-0.2999188304,
0.0126515105,
0.0953991041,
0.0301375091,
0.032952629,
0.3783244193,
0.2253104001,
-0.3717053831,
-0.3008455634,
-0.215675801,
-0.5639155507,
-0.0835940018,
0.3688941896,
0.2425529659,
-0.2383333892,
0.0979685485,
0.0944171622,
0.1586003751,
0.0331655405,
-0.1553774029,
0.1296762526,
0.3835379481,
0.1775434911,
-0.2048425376,
-0.0034462605,
0.3353902102,
-0.1584968567,
-0.1920843422,
0.1638852805,
0.0912284255,
-0.0265972074,
0.1800366342,
0.1969441622,
-0.1996732503,
0.4232635796,
0.5758476257,
0.0331580862,
0.0936800241,
-0.2577305436,
0.3264777362,
0.4559473395,
-0.039074406,
-0.180548504,
-0.1083182693,
0.1445313096,
0.1627389938,
-0.4258210063,
0.3830111027,
-0.2226231396,
-0.1333853006,
0.0844239965,
0.0172238778,
-0.5296299458,
0.0026825604,
0.1237281188,
0.18533732,
0.1231874153,
0.4071828425,
-0.0208553765,
0.3346154988,
-0.1735913754,
-0.1036755741,
0.3725515902,
-0.1448468268,
-0.2540251315,
-0.1261511594,
0.3549654186,
-0.0646672398,
-0.0190831814,
-0.3931908309,
0.1495351344,
0.4303221405,
-0.2442899495,
-0.0405435562,
0.5292306542,
-0.1951894313,
0.1532834172,
0.100050576,
0.5462212563,
0.1079476103,
-0.35732162,
-0.0870734751,
-0.2885378599
]
|
https://github.com/huggingface/datasets/issues/1992 | `datasets.map` multi processing much slower than single processing | Hi @hwijeen, you might want to look at issues #1796 and #1949. I think it could be something related to the I/O operations being performed. | Hi, thank you for the great library.
I've been using datasets to pretrain language models, and it often involves datasets as large as ~70G.
My data preparation step is roughly two steps: `load_dataset` which splits corpora into a table of sentences, and `map` converts a sentence into a list of integers, using a tokenizer.
I noticed that `map` function with `num_proc=mp.cpu_count() //2` takes more than 20 hours to finish the job where as `num_proc=1` gets the job done in about 5 hours. The machine I used has 40 cores, with 126G of RAM. There were no other jobs when `map` function was running.
What could be the reason? I would be happy to provide information necessary to spot the reason.
p.s. I was experiencing the imbalance issue mentioned in [here](https://github.com/huggingface/datasets/issues/610#issuecomment-705177036) when I was using multi processing.
p.s.2 When I run `map` with `num_proc=1`, I see one tqdm bar but all the cores are working. When `num_proc=20`, only 20 cores work.
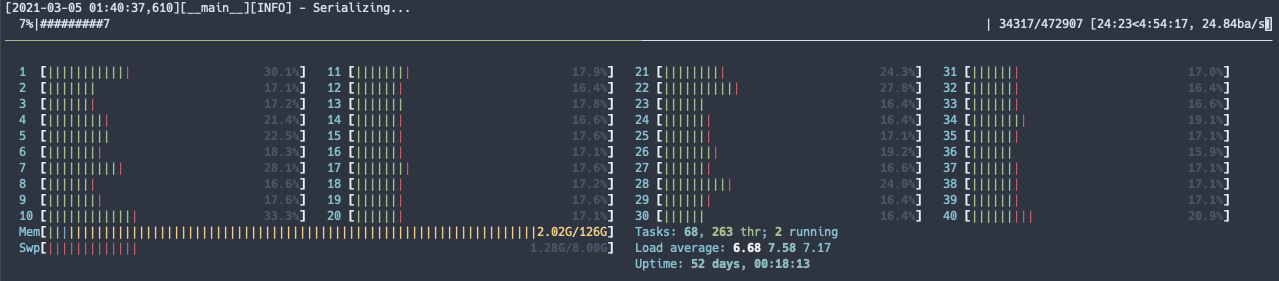
| 25 | `datasets.map` multi processing much slower than single processing
Hi, thank you for the great library.
I've been using datasets to pretrain language models, and it often involves datasets as large as ~70G.
My data preparation step is roughly two steps: `load_dataset` which splits corpora into a table of sentences, and `map` converts a sentence into a list of integers, using a tokenizer.
I noticed that `map` function with `num_proc=mp.cpu_count() //2` takes more than 20 hours to finish the job where as `num_proc=1` gets the job done in about 5 hours. The machine I used has 40 cores, with 126G of RAM. There were no other jobs when `map` function was running.
What could be the reason? I would be happy to provide information necessary to spot the reason.
p.s. I was experiencing the imbalance issue mentioned in [here](https://github.com/huggingface/datasets/issues/610#issuecomment-705177036) when I was using multi processing.
p.s.2 When I run `map` with `num_proc=1`, I see one tqdm bar but all the cores are working. When `num_proc=20`, only 20 cores work.
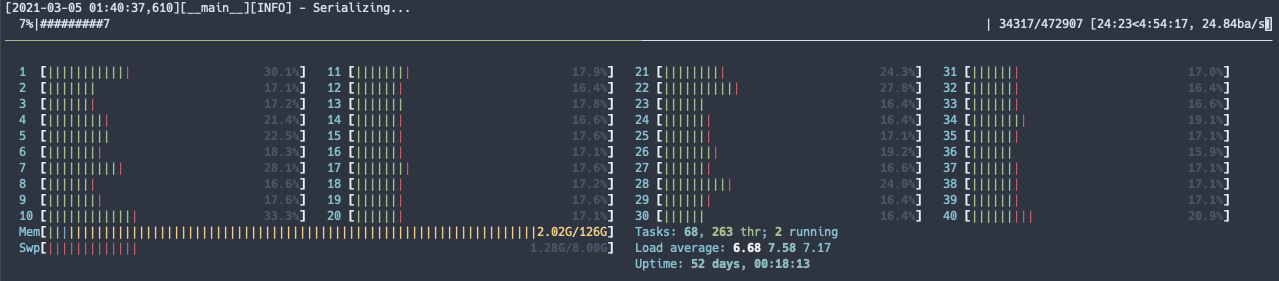
Hi @hwijeen, you might want to look at issues #1796 and #1949. I think it could be something related to the I/O operations being performed. | [
-0.4131573439,
-0.3192062378,
-0.0879166052,
0.3574213088,
-0.1027499363,
0.0203938819,
0.342405349,
0.1157952026,
0.0579246245,
-0.0040250197,
0.0656987578,
0.4142296612,
0.186817944,
0.2118219286,
-0.1675526053,
0.0080590574,
0.1905096471,
-0.0455830507,
0.174060598,
-0.0060442993,
-0.164241448,
0.1392723173,
-0.4981355667,
-0.0023557728,
-0.4168525636,
-0.1493241489,
0.1035199687,
0.1356754899,
-0.1574593484,
-0.2285334319,
-0.2626930177,
0.1599625349,
-0.0759367719,
0.5732405186,
-0.0001228117,
-0.1976779401,
0.0128010204,
0.2621523738,
0.0754681602,
0.0612592213,
-0.1325607896,
-0.2765825689,
-0.0494399518,
-0.1092961207,
0.11611332,
0.2015766799,
-0.0607616231,
-0.4477423728,
0.0259236768,
0.0857624561,
0.0549792349,
0.3612494767,
-0.3099783659,
0.0393092558,
-0.3776818812,
0.1687248349,
-0.1538439989,
0.0505479053,
0.2789545655,
-0.1809553504,
-0.1093195379,
0.3070889711,
-0.0712710321,
0.2966092825,
0.008204814,
-0.0829819292,
0.0533870496,
-0.450948149,
0.1897548139,
0.3490731716,
0.0353319719,
-0.0632035956,
-0.1647024602,
-0.2374163419,
-0.3268579543,
-0.1349691749,
0.2022649646,
0.1401261389,
0.1821073741,
-0.005169238,
-0.5538259745,
0.1473835111,
0.3451810479,
-0.07219515,
-0.1189690605,
0.0112248762,
0.12752828,
0.3288134634,
0.340477407,
0.1203623116,
-0.0500155129,
-0.220339641,
0.3193725944,
0.2178266644,
-0.7854890823,
-0.0352136604,
0.2125978917,
-0.1020860672,
-0.085489437,
-0.1859986335,
-0.1023486555,
0.3468085825,
-0.3305372894,
0.0954947472,
0.3070703745,
-0.0764188468,
0.0073989141,
0.1116777882,
-0.0347223952,
-0.1712291688,
-0.3314740658,
0.0631497875,
0.0929079652,
-0.3245914578,
-0.0648110434,
0.1292852014,
-0.3052827716,
-0.0903895423,
-0.1549665332,
0.02957597,
-0.2378289402,
-0.1023424789,
0.132678166,
0.0770516172,
-0.0129049262,
0.7939463258,
-0.2598311901,
0.1157044172,
-0.4723812044,
-0.5671404004,
-0.0263561979,
-0.1367468983,
-0.3721125424,
0.2238744348,
0.1279512346,
-0.0222435407,
0.1407320201,
0.325840354,
0.0503653511,
-0.2243560404,
0.3272612691,
-0.5305345654,
0.1972390562,
0.0816775858,
0.1585826129,
0.5495986938,
-0.1144086942,
0.3264813423,
-0.1295258552,
0.2438607812,
-0.5274050832,
-0.2451354265,
0.1805233657,
-0.0436881222,
0.1031669602,
0.1064127758,
-0.4637284875,
0.4980570674,
0.3198492229,
-0.2184123993,
-0.1693590432,
-0.1991793215,
-0.6358718276,
-0.1516771615,
-0.061387565,
0.1673316211,
-0.3309350908,
0.2380713373,
-0.3223772347,
0.146818921,
0.4350239933,
0.6122483611,
-0.1885803044,
0.3844538629,
0.0008835776,
0.1984123588,
-0.0064411908,
-0.0765073821,
-0.3745228648,
0.5169083476,
-0.1592250764,
-0.0011449485,
-0.1906939894,
0.1531788111,
0.240318805,
-0.0547439195,
0.3251419663,
0.2936647236,
0.0304951537,
0.3874754012,
-0.223006472,
-0.1107545346,
0.1460555047,
0.0392804593,
-0.0841827095,
-0.0912581757,
-0.013680188,
-0.2940004468,
0.3163228333,
-0.0127957938,
0.0252216142,
0.4302803278,
-0.3920020461,
-0.1519799978,
-0.1472371817,
-0.2302907109,
-0.0732993707,
0.3663206697,
-0.0278736558,
0.0501868241,
0.4308091402,
0.0351640098,
0.1925525963,
0.1138258651,
-0.0405157804,
-0.0642079264,
-0.1118852571,
-0.0805778205,
-0.1543218493,
-0.1789609492,
-0.009087014,
0.4500133693,
0.1817545593,
-0.1041791514,
-0.0312031191,
-0.132550776,
0.0812653452,
0.0562892854,
-0.1986993998,
-0.0696268901,
0.0854892135,
0.0502699316,
-0.0791460946,
0.2618640363,
0.4632999599,
-0.0539056994,
0.1709707677,
0.1155769825,
0.3728672862,
-0.0540256575,
0.0484472662,
-0.1458729655,
0.1021563709,
-0.23902376,
-0.023904724,
0.312074244,
0.0839070752,
0.442229718,
0.0316377841,
-0.1361157596,
-0.0101462658,
0.2965462506,
0.1542536467,
0.0568742268,
0.3458304107,
0.3308039606,
0.2301616073,
0.3350544572,
-0.1322714388,
0.3230472505,
0.6291485429,
0.0487083048,
-0.348074466,
0.043715138,
-0.1839311123,
-0.3695406616,
0.0226528011,
-0.1412670016,
0.5598791242,
0.0200403817,
0.231846422,
-0.036214184,
-0.1006811112,
-0.0587041043,
-0.0230609998,
0.102991499,
0.2264131904,
-0.1050923988,
0.295920223,
-0.0159355607,
-0.0601109527,
-0.2421923131,
0.3389693797,
0.2527020276,
-0.2600573897,
0.1031631976,
-0.2926937342,
0.1555965394,
0.1763420999,
-0.1003408656,
-0.2901909351,
-0.212841019,
-0.1558172256,
-0.0844457895,
0.2200267464,
-0.0549234226,
0.2770823836,
-0.136194095,
-0.1055421308,
-0.0475393012,
0.001006935,
-0.1382569075,
-0.1498210281,
-0.0109748095,
0.286336422,
0.2263475209,
0.041886799,
-0.0677930713,
-0.2715702653,
-0.064787291,
-0.2076480091,
-0.1236516833,
0.1410818249,
0.0555919297,
-0.193876043,
-0.0573870987,
-0.2245590389,
0.1536768973,
0.1814563721,
-0.2490201294,
-0.1899707764,
0.0516329445,
-0.106628865,
-0.2332420051,
0.0450815298,
-0.155742228,
-0.0870594904,
-0.1077109873,
0.293589592,
-0.1721996516,
0.3419691324,
-0.2260834277,
0.0634782612,
-0.0797818154,
-0.10795708,
0.0158772133,
-0.3331028819,
-0.3667623699,
-0.011990061,
-0.0184048656,
-0.1921170354,
-0.1344933659,
0.0897426903,
0.1568119377,
0.1717813313,
-0.1599654555,
0.169911921,
-0.3907405734,
0.1194333732,
0.0705733374,
0.0674809813,
0.4676004946,
0.027658863,
0.0626559779,
0.0156419929,
-0.4355050921,
-0.0635610968,
0.3192828,
0.0368365757,
0.0172181055,
0.3445577621,
0.031346038,
0.6403146982,
0.4947387576,
-0.1645862758,
0.2020887882,
0.0096634272,
-0.1716501415,
-0.4027166665,
-0.2180113494,
0.1240069866,
-0.2614231706,
0.1684180051,
0.4269154966,
0.0036421614,
-0.4294796586,
-0.0166893043,
0.2439740747,
-0.2445276678,
-0.0141027998,
0.1481050998,
-0.12326812,
0.1966448128,
0.2461673915,
-0.1976925731,
-0.351973325,
-0.0353395827,
-0.0661895424,
-0.1060340703,
-0.0986153707,
-0.2421049178,
-0.4882797599,
-0.0338982493,
-0.3716011941,
0.2611175776,
0.0420065299,
0.2969751358,
-0.0439387597,
0.1057712734,
0.3316420615,
0.0295936652,
0.5544950962,
-0.4164326489,
-0.1365627199,
0.1310192198,
-0.3980677426,
-0.2873522639,
0.0707289428,
-0.1707301587,
0.2828142941,
0.5884534121,
0.5143989325,
-0.0563279092,
-0.2053917944,
0.0086564785,
0.1212091297,
0.155375585,
-0.2661052346,
-0.3546747267,
0.1345770359,
-0.1294958442,
0.1268243641,
-0.0423873179,
0.1320044547,
0.1232841611,
-0.1420472711,
0.0194367114,
0.1154944897,
0.2381987721,
0.1704018116,
0.1206150278,
0.0930253044,
0.3004203141,
0.2673074901,
-0.0723714903,
0.0622348525,
0.3293825984,
-0.1249067858,
-0.0998571217,
0.2132406235,
0.0241131224,
0.3280751109,
0.3196834028,
0.1498576105,
0.03064996,
0.1590186805,
0.2774959505,
-0.2244988233,
0.2721707821,
0.3960228264,
0.3916811943,
-0.4936524928,
-0.5062220097,
-0.0205671154,
0.4649196267,
-0.1460789889,
0.2714833319,
-0.7679563761,
0.080063723,
0.0503084362,
0.0999302939,
0.785284996,
-0.4731327891,
0.0379718244,
-0.3119591177,
0.21543248,
-0.0481758267,
-0.5948625207,
0.1334774196,
-0.1847287714,
-0.3472977579,
0.0275630374,
-0.1090760604,
0.2031951696,
0.5102789402,
0.158322826,
0.266141355,
0.3404272497,
0.0784624442,
-0.0262438208,
0.3504179418,
0.5003808141,
-0.3187171221,
0.1645899862,
0.0327546857,
0.1939356327,
-0.207008481,
0.029859487,
0.0704518557,
-0.0611506514,
0.0293347556,
-0.3183438778,
-0.227988109,
-0.3977979422,
0.196371451,
-0.0764125213,
0.2826354504,
0.390078634,
0.2677483857,
-0.0713079423,
0.0901604742,
0.0085478863,
0.0534887426,
0.2637943327,
0.2334201038,
0.3824104965,
-0.4639773667,
-0.0287041143,
-0.1009590179,
-0.2506050467,
-0.260286361,
-0.1532425433,
-0.1185091212,
-0.122538425,
0.4491189718,
0.1657282412,
0.15709728,
-0.2264565378,
0.2328820378,
0.0790416449,
-0.1099071428,
-0.0125144245,
0.0914813429,
0.0223494954,
0.6711222529,
-0.2834817469,
-0.5138154626,
-0.0342975557,
0.3942315876,
0.2499609739,
-0.0952276289,
0.0476651937,
0.2129707634,
-0.2575140297,
-0.1355024576,
0.1535300761,
-0.1033584028,
-0.099186033,
0.1059217602,
0.0208502263,
-0.2245250791,
0.2546943426,
-0.1348887384,
-0.1512794942,
-0.0532309376,
-0.1917673647,
-0.2759398818,
-0.2528982759,
-0.1800832897,
-0.3516549766,
-0.2163471133,
0.2313815355,
0.0247281343,
0.1297440231,
0.4729891419,
-0.1329038739,
0.0583196469,
0.0901110247,
0.2110865712,
-0.0189655442,
-0.2514664531,
0.0779231042,
-0.0258276463,
-0.0658426732,
0.0795581415,
-0.0867977813,
-0.1474018842,
-0.0117546432,
0.1851729006,
-0.0224861354,
-0.2631607354,
0.3296194971,
-0.1456148773,
-0.2043029964,
-0.4302746356,
-0.0622505844,
0.0521588437,
-0.021854572,
-0.1042599678,
0.279108882,
-0.2983772457,
-0.2712825835,
0.4427228272,
-0.2003642172,
-0.0969034657,
-0.0010559582,
0.2870849669,
0.4510103464,
-0.0946666002,
-0.1005419418,
0.0290758032,
0.2644411623,
0.0692049637,
0.1374587715,
-0.0965789706,
0.181624338,
0.2666925192,
0.3389315307,
0.1272504479,
0.134645611,
-0.2531649768,
0.0023170325,
0.0016431842,
-0.2403116822,
-0.1764899343,
0.5670015216,
0.0328636207,
0.1380589008,
0.0516135283,
0.3361944258,
0.2363511771,
-0.0093843117,
-0.1060244292,
0.0810825229,
-0.2906019986,
0.0611946285,
0.1536388844,
0.2585482597,
0.2591066957,
0.4466491044,
0.1197485179,
-0.0015335807,
0.4460709393,
0.2742266059,
0.2714106143,
0.4872278273,
0.2653105855,
-0.0395573825,
-0.378324002,
0.2096998692,
0.5037137866,
-0.3329576254,
-0.0574922748,
-0.0578086153,
0.2234265059,
-0.2494558543,
-0.3842224181,
-0.2609779835,
0.3868539035,
-0.1655791402,
-0.2582349181,
0.221210286,
-0.0687718242,
-0.0023643952,
0.1776570678,
-0.2204600871,
0.1424397528,
0.7928737402,
0.130153507,
0.0441230871,
-0.3891391158,
-0.3411387205,
-0.1499899626,
0.1697581857,
-0.1381988376,
0.1828523874,
-0.3203027844,
-0.0100655295,
-0.0007450058,
0.3008361757,
0.3764934838,
0.2772648036,
-0.1428848952,
0.1006294116,
-0.0221899413,
0.1612605602,
0.0074005243,
0.1828812659,
0.0322887748,
0.1748711467,
0.1654182374,
-0.1053682193,
-0.0901593417,
-0.4533829391,
0.0302178673,
0.353749454,
-0.1493939161,
0.1578142941,
-0.3038738668,
-0.1554051638,
0.0109656481,
0.2941297293,
-0.2735160589,
-0.0898392126,
0.4953569174,
-0.4910948277,
0.0730638951,
-0.151382342,
0.0253127664,
-0.0798001885,
0.5650861263,
0.3381140232,
0.010556587,
-0.4115411639,
-0.1151146367,
-0.5973331928,
-0.0711950511,
-0.3206049204,
0.2698099315,
0.0732985362,
0.2909342945,
0.0744077191,
0.121265091,
0.4038817585,
-0.0933897421,
-0.0835427642,
0.3543794155,
-0.3133423924,
0.4769581854,
-0.243443355,
-0.1946043521,
-0.0849100947,
-0.4186907411,
0.3781763315,
0.0753600374,
-0.1486231089,
-0.1737610251,
-0.0027934471,
0.1544392407,
0.147845,
0.1885034144,
0.3548044562,
0.3634969592,
0.0412944928,
0.0078074099,
-0.1190248728,
0.1936976463,
-0.0414555669,
0.3311105967,
-0.0583353154,
0.0689005852,
-0.3055815995,
0.1666467339,
0.1130412221,
-0.1093769148,
0.0038677147,
-0.2370978892,
-0.3271935582,
-0.0505088978,
-0.0389762819,
0.3171595931,
0.095273979,
0.4033237696,
0.1399893761,
-0.0934453011,
-0.1850920916,
-0.0801377818,
-0.028804604,
-0.266825527,
-0.290576905,
-0.4305557907,
0.2277180105,
-0.2528908849,
0.1613215655,
-0.2331477553,
-0.3237471879,
0.3040857017,
-0.0249335859,
-0.3681617975,
0.3654677868,
-0.3870626986,
-0.0856688619,
-0.1473060846,
0.0290636159,
-0.0981447473,
-0.4448870122,
0.1913252771,
-0.1197810695
]
|
https://github.com/huggingface/datasets/issues/1992 | `datasets.map` multi processing much slower than single processing | I see that many people are experiencing the same issue. Is this problem considered an "official" bug that is worth a closer look? @lhoestq | Hi, thank you for the great library.
I've been using datasets to pretrain language models, and it often involves datasets as large as ~70G.
My data preparation step is roughly two steps: `load_dataset` which splits corpora into a table of sentences, and `map` converts a sentence into a list of integers, using a tokenizer.
I noticed that `map` function with `num_proc=mp.cpu_count() //2` takes more than 20 hours to finish the job where as `num_proc=1` gets the job done in about 5 hours. The machine I used has 40 cores, with 126G of RAM. There were no other jobs when `map` function was running.
What could be the reason? I would be happy to provide information necessary to spot the reason.
p.s. I was experiencing the imbalance issue mentioned in [here](https://github.com/huggingface/datasets/issues/610#issuecomment-705177036) when I was using multi processing.
p.s.2 When I run `map` with `num_proc=1`, I see one tqdm bar but all the cores are working. When `num_proc=20`, only 20 cores work.
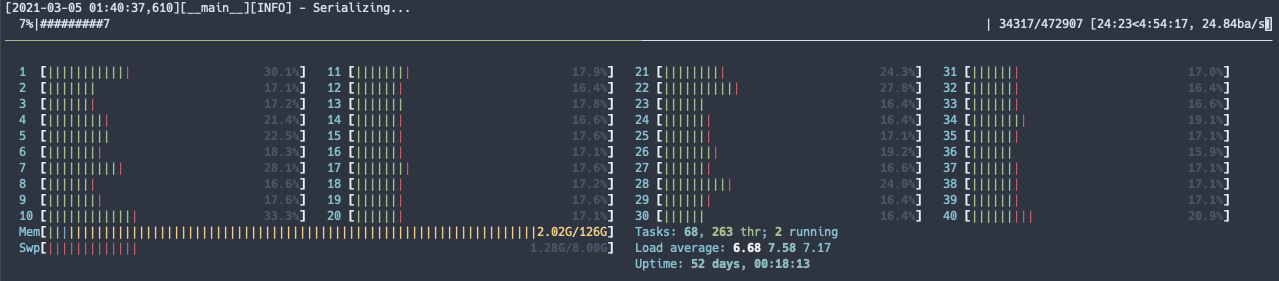
| 24 | `datasets.map` multi processing much slower than single processing
Hi, thank you for the great library.
I've been using datasets to pretrain language models, and it often involves datasets as large as ~70G.
My data preparation step is roughly two steps: `load_dataset` which splits corpora into a table of sentences, and `map` converts a sentence into a list of integers, using a tokenizer.
I noticed that `map` function with `num_proc=mp.cpu_count() //2` takes more than 20 hours to finish the job where as `num_proc=1` gets the job done in about 5 hours. The machine I used has 40 cores, with 126G of RAM. There were no other jobs when `map` function was running.
What could be the reason? I would be happy to provide information necessary to spot the reason.
p.s. I was experiencing the imbalance issue mentioned in [here](https://github.com/huggingface/datasets/issues/610#issuecomment-705177036) when I was using multi processing.
p.s.2 When I run `map` with `num_proc=1`, I see one tqdm bar but all the cores are working. When `num_proc=20`, only 20 cores work.
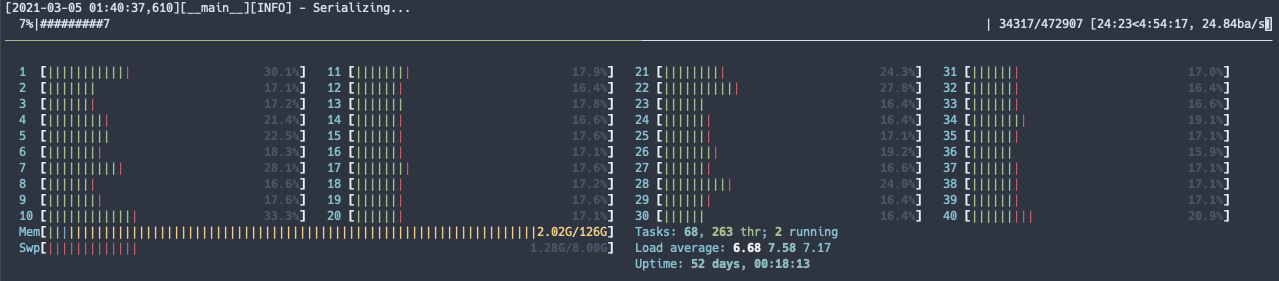
I see that many people are experiencing the same issue. Is this problem considered an "official" bug that is worth a closer look? @lhoestq | [
-0.3866334856,
-0.2725814879,
-0.0787934363,
0.3493686914,
-0.1060923487,
-0.0051659779,
0.3705374002,
0.1268498451,
0.0547807962,
-0.016425699,
0.0762920827,
0.4323449731,
0.1645939946,
0.1775635779,
-0.1425019652,
0.0690408275,
0.2205798924,
-0.0627334192,
0.1990935504,
-0.0131207593,
-0.1687244922,
0.1928427219,
-0.4963890612,
-0.0021658849,
-0.4106544256,
-0.1248213127,
0.1040454581,
0.1048686132,
-0.1357279122,
-0.2046504915,
-0.2140672058,
0.1370119452,
-0.135569185,
0.5738761425,
-0.0001229708,
-0.1984732598,
0.054988049,
0.2633066475,
0.0764160678,
0.0830864459,
-0.1329541355,
-0.2601751089,
-0.0424125306,
-0.0829151496,
0.1244728863,
0.2161737829,
-0.058563754,
-0.4646045268,
-0.0096626692,
0.084335424,
0.0631441101,
0.3764816225,
-0.2817438841,
0.0143860234,
-0.3346053362,
0.1581187397,
-0.1740892082,
0.0507871881,
0.2852862477,
-0.1864518076,
-0.1175593808,
0.3048100173,
-0.0474988595,
0.3209557235,
0.0011853832,
-0.0322241783,
0.0270202942,
-0.4442290366,
0.162076667,
0.3706926107,
0.0055630566,
-0.072739698,
-0.1854883581,
-0.2690019906,
-0.3177760243,
-0.1561565399,
0.2311845422,
0.1288771629,
0.1493100226,
-0.0132250581,
-0.5340878367,
0.1374521405,
0.3866600692,
-0.0713502467,
-0.1523336768,
0.0201122221,
0.1103037372,
0.309466511,
0.3142452538,
0.1228396073,
-0.0455446132,
-0.1715077013,
0.2885458171,
0.1829547435,
-0.7955271602,
-0.0417100899,
0.2489287555,
-0.1129600629,
-0.0615522377,
-0.1814658195,
-0.0985891819,
0.3503520787,
-0.3340126574,
0.1081216335,
0.3353156447,
-0.0971494541,
0.0022065956,
0.0807370469,
0.0160702653,
-0.164410606,
-0.3038091958,
0.1016725674,
0.1055112481,
-0.3424448073,
-0.0712612197,
0.1095167324,
-0.2824555337,
-0.0873279348,
-0.1478879899,
0.0589938723,
-0.2159599364,
-0.0632728487,
0.1097482145,
0.0793706551,
-0.01925111,
0.8041567206,
-0.2690223157,
0.0870177597,
-0.4744414091,
-0.5820880532,
-0.018575022,
-0.1468961984,
-0.3591332436,
0.1967960149,
0.1042193845,
-0.0287771188,
0.1002884507,
0.3114528656,
0.0865714327,
-0.2278884798,
0.3016510606,
-0.4975842237,
0.2251565903,
0.1189087927,
0.1471591294,
0.5624079108,
-0.1207758561,
0.3020248115,
-0.1247039884,
0.2404896617,
-0.5386422873,
-0.2262618244,
0.1390105784,
-0.0469703786,
0.0906337649,
0.1150419638,
-0.4588498473,
0.4970787466,
0.3395266235,
-0.2471322566,
-0.1647281945,
-0.1944393367,
-0.6674044728,
-0.1534183025,
-0.0376288481,
0.1841218174,
-0.3353409171,
0.2206213623,
-0.3466390669,
0.1560325772,
0.4348998368,
0.6155385375,
-0.1899300069,
0.3575473428,
-0.0506501123,
0.202154398,
-0.0163860694,
-0.0397708975,
-0.3898436427,
0.536513865,
-0.1695720702,
0.0112686465,
-0.225787878,
0.1606897861,
0.2166396379,
-0.060812559,
0.3597381115,
0.2520618737,
0.0037233683,
0.4067164361,
-0.2268608361,
-0.148475796,
0.1806095243,
0.0356507413,
-0.050955683,
-0.1092447415,
-0.0207678769,
-0.2912406027,
0.3273308873,
0.0346137062,
0.0190519728,
0.4201274514,
-0.3767902851,
-0.1781182885,
-0.1492094845,
-0.2470978051,
-0.0693646222,
0.3475501537,
-0.0082943439,
0.0345355012,
0.4530821443,
0.0276289769,
0.2079562247,
0.1241190359,
-0.0410280004,
-0.0788128525,
-0.1196677983,
-0.0874338225,
-0.1914295703,
-0.1583939344,
-0.0411384441,
0.451487422,
0.2057487965,
-0.1229941845,
-0.0540046245,
-0.1302856058,
0.0977362022,
0.0768275857,
-0.1990161389,
-0.0822813511,
0.0642762631,
0.027988337,
-0.0868976116,
0.2680095136,
0.4869925976,
-0.0700862855,
0.1350849271,
0.087985076,
0.40506199,
-0.0231115706,
-0.0172425397,
-0.1115204021,
0.1151866317,
-0.2409276366,
-0.0333325006,
0.3163408339,
0.1158864722,
0.399556756,
0.0307293087,
-0.1051460728,
0.0267667491,
0.2851706147,
0.1078849509,
0.0460049585,
0.3108840883,
0.3136244714,
0.2151769996,
0.320510149,
-0.1061689928,
0.3456169069,
0.6657005548,
0.0833152756,
-0.3104914129,
0.0496395677,
-0.1518193632,
-0.3581571281,
0.014146057,
-0.1226073578,
0.5757824779,
0.0022506306,
0.2472877949,
-0.0382557064,
-0.1148133725,
-0.080982767,
-0.0141079519,
0.1080095023,
0.2107910961,
-0.0710329041,
0.3324417472,
-0.0037923008,
-0.1000335738,
-0.2148347497,
0.3497828245,
0.2076939791,
-0.2833828032,
0.1074734926,
-0.3034692705,
0.2022359073,
0.1599316746,
-0.1036075503,
-0.301364243,
-0.2368096113,
-0.1221836805,
-0.0886194706,
0.1853171587,
-0.0142415175,
0.2501137257,
-0.0963029116,
-0.1153763384,
-0.0365736261,
0.0350124724,
-0.1128342897,
-0.1560256034,
-0.0114225699,
0.2804484665,
0.1901464909,
0.0729655176,
-0.0563282855,
-0.277739495,
-0.1246763244,
-0.24367553,
-0.0937551707,
0.1492809504,
0.0766108334,
-0.1636892408,
-0.1074148118,
-0.207832858,
0.1690099835,
0.1589676738,
-0.3020037413,
-0.2018778324,
0.0461155511,
-0.1185490489,
-0.2314852327,
-0.0106225777,
-0.1588874757,
-0.0949647054,
-0.1099473387,
0.2879396081,
-0.1672471762,
0.3199920058,
-0.1932986975,
0.0527213924,
-0.0889679715,
-0.1160117686,
-0.042556107,
-0.3604643345,
-0.3457469344,
-0.0074075204,
-0.0419457927,
-0.2252166122,
-0.1405960321,
0.0771877244,
0.1546164453,
0.1581597328,
-0.1823361516,
0.174954325,
-0.3803263903,
0.1397096217,
0.0869093984,
0.0220158827,
0.4934561849,
-0.0037511857,
0.0688595548,
0.0072935456,
-0.4403318167,
-0.0629736185,
0.2977602184,
0.0230549723,
0.0195934474,
0.351814568,
0.0513638519,
0.6315265894,
0.4889098108,
-0.150758177,
0.1987278163,
-0.0477828495,
-0.1115793213,
-0.3996534348,
-0.2119542211,
0.1117120311,
-0.2826230824,
0.1254272461,
0.4168573618,
-0.0117393108,
-0.4363336265,
-0.0093131755,
0.2886064351,
-0.2262657434,
-0.0335346796,
0.1407322139,
-0.1364982724,
0.1556614041,
0.2492787838,
-0.1519769132,
-0.3358055651,
-0.0443012565,
-0.0655483305,
-0.1016887724,
-0.1039938629,
-0.275454998,
-0.4782882929,
-0.0116194393,
-0.3779992163,
0.2300856411,
0.0478085279,
0.3498313725,
-0.0442247503,
0.0869575217,
0.3212326169,
-0.0168988537,
0.5688454509,
-0.3910735548,
-0.1088192612,
0.1516806483,
-0.359442085,
-0.288334161,
0.0306874979,
-0.1507096142,
0.2883811891,
0.6098183393,
0.5065738559,
-0.0416914895,
-0.1537957788,
0.0076691383,
0.0976756737,
0.1281902492,
-0.2506243885,
-0.3729522526,
0.1217524335,
-0.0959061235,
0.1306333989,
0.0143189644,
0.1115721837,
0.1250598431,
-0.1616068631,
0.0231330376,
0.0852763727,
0.2221222073,
0.1544778198,
0.1185790151,
0.0978062972,
0.3397477567,
0.2531830966,
-0.0906121209,
0.0212657601,
0.3452210426,
-0.1188253686,
-0.1019111276,
0.2752825618,
0.0147785507,
0.3062776327,
0.3589981198,
0.1124793142,
0.0289851706,
0.1859193742,
0.26042521,
-0.2158242613,
0.2447052002,
0.4137661159,
0.3523692489,
-0.4705944955,
-0.4870201647,
-0.0180302896,
0.4810300469,
-0.1415035725,
0.3186241686,
-0.7869359851,
0.0805434808,
0.0308837965,
0.0685206354,
0.7341236472,
-0.4244520366,
0.084488526,
-0.2732584476,
0.2042400539,
-0.0453797355,
-0.5877479911,
0.1424937248,
-0.1993722767,
-0.3385687172,
0.0265435521,
-0.1084180549,
0.1729332209,
0.4824617803,
0.1901150197,
0.2567996681,
0.3360640407,
0.1500345916,
-0.0127168149,
0.3075158596,
0.4955806434,
-0.3149343729,
0.1551858634,
0.0297930799,
0.2141150236,
-0.1911161989,
0.0350001827,
0.0768580139,
-0.045210816,
-0.0057106297,
-0.3479852974,
-0.1982783228,
-0.3737333417,
0.2166281343,
-0.0152998995,
0.2827971578,
0.3574750423,
0.2437444776,
-0.059813153,
0.0877164677,
-0.0267882459,
0.0855960324,
0.2774122655,
0.2729421556,
0.3718232512,
-0.4484906197,
-0.0329685062,
-0.0818325803,
-0.1895796061,
-0.2662755251,
-0.1657214612,
-0.1383871734,
-0.1347094029,
0.5105387568,
0.1755461544,
0.1854098141,
-0.1983138472,
0.2382969111,
0.1368565261,
-0.1418379247,
-0.0154677751,
0.0910694227,
0.0367256254,
0.6409505606,
-0.3012273908,
-0.4983737171,
-0.0441618748,
0.4551080167,
0.2364107072,
-0.0826209188,
0.0378698111,
0.2227641493,
-0.2593607306,
-0.1275499314,
0.1750159562,
-0.1204334497,
-0.1331340969,
0.142290473,
-0.0169947241,
-0.2429298013,
0.2397280335,
-0.1239748299,
-0.1485930979,
-0.0695603937,
-0.1981938928,
-0.2745432854,
-0.2284981012,
-0.1922996044,
-0.3533956409,
-0.2301903516,
0.2255143374,
0.027039757,
0.1312207282,
0.4111308753,
-0.1242665872,
0.0634134859,
0.1151674092,
0.1511101872,
-0.0477394722,
-0.2192235142,
0.0848912075,
-0.0454672314,
-0.088640511,
0.1240957975,
-0.0863494873,
-0.1349318177,
-0.0359488502,
0.1907496601,
-0.0293509848,
-0.2500730455,
0.3638283312,
-0.1229364574,
-0.2473055422,
-0.4124503732,
-0.0434983708,
0.0781403184,
-0.0084608458,
-0.1043872461,
0.2735492587,
-0.3038493693,
-0.2333778292,
0.4588102698,
-0.183139354,
-0.0493290909,
-0.0164840892,
0.2902966738,
0.441370368,
-0.0644909218,
-0.1011531204,
0.027640041,
0.2080293894,
0.0845933408,
0.1611506492,
-0.1326497048,
0.1946247071,
0.2788704038,
0.3437086642,
0.1637817919,
0.073567085,
-0.2542277873,
-0.0023205944,
-0.0041939439,
-0.2574893832,
-0.2087834626,
0.5838805437,
0.096436061,
0.1197779104,
0.0411368422,
0.361236304,
0.186149925,
-0.0093718013,
-0.0711821541,
0.0869952664,
-0.3279542029,
0.0698114559,
0.1804552823,
0.2434645444,
0.29932338,
0.427968055,
0.0917912349,
0.0042310609,
0.4806730747,
0.2743299603,
0.25569731,
0.5029738545,
0.2437454611,
-0.0248369072,
-0.3844451904,
0.2235190868,
0.4877792001,
-0.3467883766,
-0.0386702642,
-0.0356296189,
0.2398197949,
-0.275962472,
-0.3680636585,
-0.3111706674,
0.3639386296,
-0.1686622202,
-0.2518335879,
0.2508213222,
-0.0536626317,
0.0110086948,
0.1883884519,
-0.207102865,
0.1562433839,
0.8072531223,
0.1221695542,
0.0070221513,
-0.3940957487,
-0.3178668916,
-0.1933318228,
0.1779832095,
-0.1600326449,
0.1832062304,
-0.3481115997,
-0.0136615746,
-0.0037102741,
0.3205559552,
0.3718772531,
0.288512826,
-0.130416736,
0.047796905,
-0.0299580712,
0.1783383489,
-0.0260137878,
0.2189337462,
0.0283294618,
0.1776463836,
0.1494836509,
-0.1087213531,
-0.0802765638,
-0.3959597647,
0.0510726236,
0.392252326,
-0.1891976446,
0.1715061963,
-0.3878602982,
-0.1462849528,
0.0034629845,
0.2993425131,
-0.2532043755,
-0.1017914191,
0.5167979002,
-0.4817173779,
0.0881935582,
-0.1758194417,
0.0260637794,
-0.0718232021,
0.5631068349,
0.2815415859,
-0.0157233607,
-0.3569283485,
-0.0953781456,
-0.6198185086,
-0.0195464846,
-0.3267098367,
0.3234164715,
0.0649850816,
0.3096296787,
0.0509841703,
0.1159685403,
0.3893098831,
-0.1246113554,
-0.0964445099,
0.3271768689,
-0.3246978223,
0.4432680309,
-0.223360911,
-0.1983082443,
-0.0727174133,
-0.4446119964,
0.3880726993,
0.054356277,
-0.1546517164,
-0.1481701732,
-0.059766233,
0.1554164141,
0.1724304408,
0.189253971,
0.3503004909,
0.3331795633,
0.0380780138,
-0.0212680344,
-0.165464595,
0.1553389728,
-0.0420161262,
0.3519108295,
-0.0852080286,
0.0682391152,
-0.2896392345,
0.1777037233,
0.0935720652,
-0.1146297678,
0.0163654685,
-0.201313436,
-0.3573484123,
-0.0273928046,
-0.0389279239,
0.3227158189,
0.0799016207,
0.3904701471,
0.0798128098,
-0.0845014155,
-0.1620465964,
-0.1161689982,
-0.0229115169,
-0.3266755044,
-0.2992131412,
-0.4464147091,
0.2472546399,
-0.2571527362,
0.1094823107,
-0.2349526435,
-0.3330852091,
0.3025572896,
0.0018812267,
-0.3634395003,
0.3403201401,
-0.4263150692,
-0.0976774767,
-0.1567449719,
0.0731857494,
-0.0885367915,
-0.4450535774,
0.2454165965,
-0.1163509935
]
|
https://github.com/huggingface/datasets/issues/1992 | `datasets.map` multi processing much slower than single processing | Yes this is an official bug. On my side I haven't managed to reproduce it but @theo-m has. We'll investigate this ! | Hi, thank you for the great library.
I've been using datasets to pretrain language models, and it often involves datasets as large as ~70G.
My data preparation step is roughly two steps: `load_dataset` which splits corpora into a table of sentences, and `map` converts a sentence into a list of integers, using a tokenizer.
I noticed that `map` function with `num_proc=mp.cpu_count() //2` takes more than 20 hours to finish the job where as `num_proc=1` gets the job done in about 5 hours. The machine I used has 40 cores, with 126G of RAM. There were no other jobs when `map` function was running.
What could be the reason? I would be happy to provide information necessary to spot the reason.
p.s. I was experiencing the imbalance issue mentioned in [here](https://github.com/huggingface/datasets/issues/610#issuecomment-705177036) when I was using multi processing.
p.s.2 When I run `map` with `num_proc=1`, I see one tqdm bar but all the cores are working. When `num_proc=20`, only 20 cores work.
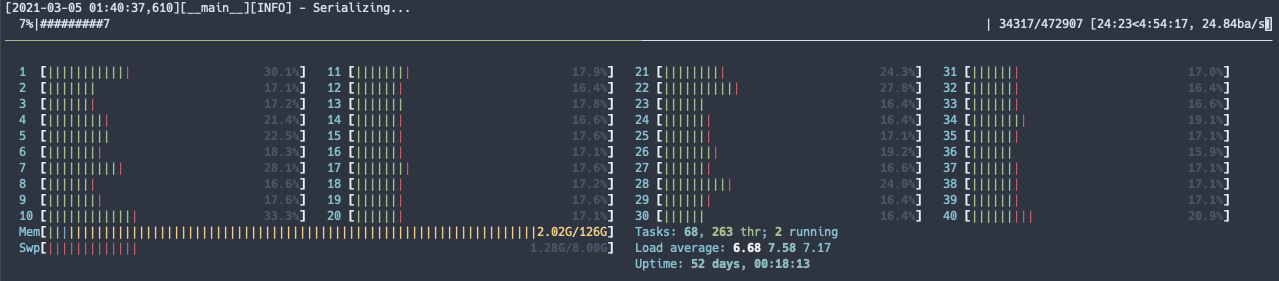
| 22 | `datasets.map` multi processing much slower than single processing
Hi, thank you for the great library.
I've been using datasets to pretrain language models, and it often involves datasets as large as ~70G.
My data preparation step is roughly two steps: `load_dataset` which splits corpora into a table of sentences, and `map` converts a sentence into a list of integers, using a tokenizer.
I noticed that `map` function with `num_proc=mp.cpu_count() //2` takes more than 20 hours to finish the job where as `num_proc=1` gets the job done in about 5 hours. The machine I used has 40 cores, with 126G of RAM. There were no other jobs when `map` function was running.
What could be the reason? I would be happy to provide information necessary to spot the reason.
p.s. I was experiencing the imbalance issue mentioned in [here](https://github.com/huggingface/datasets/issues/610#issuecomment-705177036) when I was using multi processing.
p.s.2 When I run `map` with `num_proc=1`, I see one tqdm bar but all the cores are working. When `num_proc=20`, only 20 cores work.
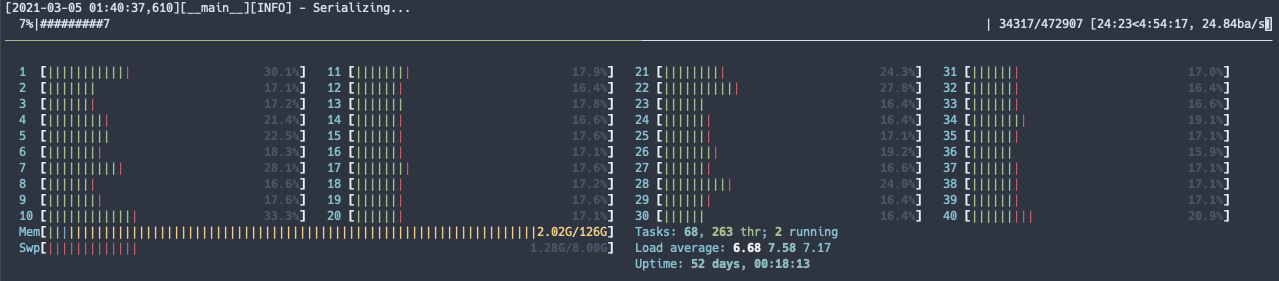
Yes this is an official bug. On my side I haven't managed to reproduce it but @theo-m has. We'll investigate this ! | [
-0.4096489549,
-0.3040941954,
-0.0847012475,
0.3398915231,
-0.0897001252,
0.0127339009,
0.352761507,
0.1181462258,
0.0582123809,
0.0094827693,
0.0727025419,
0.4454631209,
0.1630734205,
0.1893293262,
-0.1400845945,
0.0302212369,
0.2145637274,
-0.0554910563,
0.1930124462,
-0.0090902559,
-0.1625764817,
0.15560247,
-0.5023615956,
0.0061128489,
-0.4096406698,
-0.1224155352,
0.1134263277,
0.1184024438,
-0.1367545873,
-0.2299796939,
-0.23569116,
0.1276424676,
-0.0973549262,
0.5718789697,
-0.000123495,
-0.1887064427,
0.0392466038,
0.2753136456,
0.0935694575,
0.074359104,
-0.139945209,
-0.2675496936,
-0.0483152196,
-0.0967940614,
0.1221138462,
0.2130556256,
-0.0675205812,
-0.4581288695,
-0.0038365512,
0.0869870782,
0.0545999631,
0.3697356582,
-0.3081155121,
0.0285560284,
-0.3477621973,
0.1482301056,
-0.1653359681,
0.0483659543,
0.2727541924,
-0.1806853116,
-0.1124006435,
0.3173141181,
-0.0748665929,
0.3036651611,
-0.0166221987,
-0.0566673242,
0.0528344773,
-0.4617329538,
0.184114188,
0.3488666117,
0.0046462342,
-0.0733980164,
-0.1476433575,
-0.2400643826,
-0.3316353261,
-0.1445307136,
0.2060097754,
0.1393591911,
0.170795992,
-0.003037418,
-0.5398433208,
0.1457113177,
0.356788516,
-0.0727699474,
-0.1326306164,
0.0157897081,
0.1214101166,
0.3247835636,
0.329547286,
0.1282670796,
-0.0393195823,
-0.1861978322,
0.3062700629,
0.2130704373,
-0.7936055064,
-0.0484880544,
0.2307212353,
-0.1161448061,
-0.0666977242,
-0.1693448275,
-0.1044374928,
0.3401470482,
-0.3380641639,
0.1036484614,
0.2965789437,
-0.0940638632,
0.0115038976,
0.1055323035,
-0.0219706874,
-0.1720060706,
-0.3138700724,
0.0885759965,
0.1108503416,
-0.3309872448,
-0.0520169251,
0.1264753342,
-0.2921320796,
-0.0832153782,
-0.1314231455,
0.0433556549,
-0.2141341567,
-0.0657923669,
0.1219368502,
0.0876592994,
-0.0205291081,
0.8091930151,
-0.2801373005,
0.1047494039,
-0.4791384339,
-0.5906726122,
-0.0183127187,
-0.1310724318,
-0.3497511148,
0.2159639597,
0.1177537292,
-0.0320881791,
0.1305217296,
0.3339615762,
0.0465602465,
-0.2266204059,
0.3357835412,
-0.5207980871,
0.2082928717,
0.1060205847,
0.1533148438,
0.5560805202,
-0.1143065318,
0.310634762,
-0.1215612292,
0.2645983398,
-0.5323987007,
-0.2379590422,
0.144897148,
-0.0522092134,
0.1052638516,
0.1218637154,
-0.4693042934,
0.4962762892,
0.3239688873,
-0.2415211201,
-0.1572943181,
-0.1935510486,
-0.6807103157,
-0.156635195,
-0.048270043,
0.1644488424,
-0.3335205317,
0.2270423174,
-0.3317821622,
0.1459441334,
0.4463774562,
0.5977522731,
-0.1945760101,
0.3576442301,
-0.023772344,
0.1998114437,
-0.012190056,
-0.059124209,
-0.3943871856,
0.5213027,
-0.1812318563,
-0.0069302963,
-0.2187444419,
0.1681262404,
0.2308541089,
-0.058527261,
0.3284296393,
0.274037689,
0.0182396416,
0.4006505013,
-0.2312323153,
-0.1404779404,
0.1745203435,
0.0220592413,
-0.0664570406,
-0.0973710343,
-0.0106212813,
-0.2823181748,
0.3429298997,
0.0160741191,
0.0190717541,
0.4239673913,
-0.383946985,
-0.1766142398,
-0.1408476084,
-0.2507998049,
-0.0771213174,
0.3548489213,
-0.0161996856,
0.0330528244,
0.4360340536,
0.0295184124,
0.1947902739,
0.1215819418,
-0.0341642126,
-0.0828239247,
-0.1245476082,
-0.0914572999,
-0.1777032316,
-0.1682780385,
-0.0154164964,
0.4407098591,
0.20545654,
-0.113658309,
-0.0448654033,
-0.1442040354,
0.0908378735,
0.095868893,
-0.2234877795,
-0.0967265069,
0.0980234742,
0.0393654257,
-0.0858788639,
0.2614977062,
0.4735928476,
-0.0647705048,
0.1389828622,
0.0886042565,
0.381934464,
-0.051806014,
0.0125041027,
-0.1227143928,
0.1179006398,
-0.2445403486,
-0.017745804,
0.3030709028,
0.1027350724,
0.4237642884,
0.0093256002,
-0.1245472059,
0.0180588048,
0.2912707329,
0.1467741728,
0.0439319313,
0.3255983293,
0.3314090967,
0.2144908309,
0.3231861889,
-0.1254150271,
0.3435010314,
0.6361767054,
0.0632758439,
-0.3252545297,
0.031858474,
-0.1783329099,
-0.358253628,
0.0271827038,
-0.1357809901,
0.5621808767,
0.0008782006,
0.2314520031,
-0.0275594853,
-0.1016285196,
-0.0770716667,
-0.0270146355,
0.1100120023,
0.2270743698,
-0.1055658236,
0.3235616386,
-0.0128857065,
-0.0454218015,
-0.2321379185,
0.3384540081,
0.2258009315,
-0.2655063272,
0.1165241897,
-0.3123520911,
0.1925365031,
0.1699938625,
-0.1106920913,
-0.2881995738,
-0.2154275626,
-0.1364983171,
-0.0801258385,
0.1979136765,
-0.0297544301,
0.259024471,
-0.1393478811,
-0.1104808599,
-0.0400148928,
0.0211553685,
-0.1237186939,
-0.1452608258,
-0.0168918427,
0.274161309,
0.208908543,
0.0379454531,
-0.0567799658,
-0.2843345404,
-0.0929553434,
-0.2236782908,
-0.1036629602,
0.1599895358,
0.0655344203,
-0.1882635653,
-0.0869258717,
-0.2204575688,
0.1812091321,
0.1581974775,
-0.2650476396,
-0.1830224246,
0.06016526,
-0.1190668494,
-0.2122449577,
0.0102416808,
-0.1542368829,
-0.0883427635,
-0.092578195,
0.2850443423,
-0.1693925261,
0.343277514,
-0.2147858888,
0.062860854,
-0.086412333,
-0.1067369282,
-0.0121780336,
-0.3401825428,
-0.3741227388,
-0.0052902861,
-0.0206396785,
-0.2117352337,
-0.1438672394,
0.0744258687,
0.1531663537,
0.1476217657,
-0.173732847,
0.1924839616,
-0.392159611,
0.1361327469,
0.0613378771,
0.026609648,
0.4794920385,
0.0184000339,
0.0740957335,
0.0087159555,
-0.4381611943,
-0.0841047391,
0.2906068265,
0.0134119838,
0.0183812939,
0.3479087651,
0.0413983464,
0.6347274184,
0.4861660004,
-0.1431153715,
0.1921540052,
-0.024266785,
-0.1544579417,
-0.3937439024,
-0.2161060572,
0.1225519925,
-0.262909472,
0.1614924818,
0.410754621,
-0.0043057911,
-0.4227928817,
-0.0008047907,
0.2321251482,
-0.2147951275,
-0.0154876253,
0.1425656378,
-0.1290990263,
0.1569970548,
0.250865519,
-0.1738856286,
-0.347938776,
-0.0462886393,
-0.0596803166,
-0.1070539057,
-0.1073272377,
-0.2466082722,
-0.4860389829,
-0.0262888782,
-0.3734413683,
0.2421614826,
0.0494489372,
0.3200061321,
-0.0182063244,
0.1125688553,
0.3193677962,
0.013595473,
0.5667481422,
-0.3824682534,
-0.1051651984,
0.1393255293,
-0.369050324,
-0.2961440384,
0.0472288541,
-0.1584997922,
0.2740091383,
0.5899749994,
0.5148351192,
-0.0092027998,
-0.1744456738,
-0.0026542204,
0.1248296201,
0.1343102008,
-0.2558061182,
-0.3702331483,
0.1224806607,
-0.1186218187,
0.131118238,
-0.0073252968,
0.1288422197,
0.1130924821,
-0.1465183794,
0.025368819,
0.0986625701,
0.205263555,
0.1549216956,
0.1151605248,
0.0833991244,
0.3108261824,
0.2329062372,
-0.0853873342,
0.0293090902,
0.3309388161,
-0.1140492484,
-0.0981436968,
0.2457461804,
0.0296435524,
0.3158793449,
0.3062439263,
0.1195378974,
0.0503817201,
0.1765029579,
0.2736958861,
-0.2165957093,
0.2651928067,
0.4047733545,
0.3798927665,
-0.475181073,
-0.4996890426,
-0.0021379946,
0.4812621176,
-0.1455397606,
0.2885550857,
-0.8008272648,
0.0941816047,
0.0404599383,
0.0750562921,
0.7513073087,
-0.4283935726,
0.0547339357,
-0.2906383872,
0.1864209175,
-0.0401574895,
-0.5946905017,
0.1480189115,
-0.1938690394,
-0.3592973351,
0.026049966,
-0.1081056297,
0.1894502342,
0.4942733049,
0.1704991311,
0.2647926509,
0.3171116412,
0.1156070456,
-0.0115670795,
0.3212321997,
0.5124132633,
-0.3162501454,
0.1704144627,
0.0245616045,
0.2073134333,
-0.2166734636,
0.0332605913,
0.0919921473,
-0.0394996069,
0.0051119165,
-0.3249229491,
-0.1912872493,
-0.3838327527,
0.2179379314,
-0.0508309603,
0.2920315564,
0.3759082258,
0.2679612041,
-0.0715066269,
0.0968254209,
0.0025583298,
0.0619819164,
0.2923752666,
0.2626042962,
0.3842225969,
-0.4712411761,
-0.0278316867,
-0.1048300043,
-0.2401940972,
-0.25318259,
-0.1586776376,
-0.120327808,
-0.1395846307,
0.4965133071,
0.1751246154,
0.190396145,
-0.2085006386,
0.2218901366,
0.1242449507,
-0.1349463612,
-0.0184658226,
0.0999104381,
0.0267607905,
0.6535869241,
-0.310385704,
-0.5013617873,
-0.0315314569,
0.4279741347,
0.2679143548,
-0.0882949606,
0.0311931744,
0.2301477939,
-0.2587218285,
-0.1286641955,
0.1715879738,
-0.1222177371,
-0.1143977195,
0.1223205924,
-0.0090483651,
-0.228537932,
0.2377549261,
-0.1473871917,
-0.1642848998,
-0.0562660769,
-0.195502758,
-0.279969871,
-0.2492466122,
-0.1993587613,
-0.3673151731,
-0.2489990145,
0.2481583506,
0.0355370417,
0.1180195138,
0.4483624697,
-0.1203230992,
0.0711580515,
0.1135154292,
0.180223763,
-0.0537471175,
-0.2146888822,
0.0826704502,
-0.0330272093,
-0.0839464441,
0.1185898706,
-0.082695201,
-0.137053743,
-0.0379953757,
0.1925502419,
-0.0232910737,
-0.2450544834,
0.341016233,
-0.1211562455,
-0.2160391062,
-0.408619374,
-0.0402072966,
0.070365347,
0.0017751751,
-0.1067221314,
0.2822425067,
-0.3028360605,
-0.263941735,
0.4480732083,
-0.190210402,
-0.0775017887,
-0.0155576291,
0.2981881499,
0.4519785941,
-0.080932878,
-0.0976521,
0.019575933,
0.2365053296,
0.0848744884,
0.1398589313,
-0.119976379,
0.1827152818,
0.262699008,
0.3164889514,
0.1239286065,
0.0985501781,
-0.2516514361,
0.0024662295,
-0.0108008832,
-0.2553519309,
-0.2139280438,
0.5657917261,
0.0606563166,
0.1268815547,
0.0375495106,
0.3244930804,
0.205741778,
-0.0158213731,
-0.0779163167,
0.0725621805,
-0.3115457594,
0.0609444641,
0.1631502956,
0.2488365322,
0.2783690989,
0.4251826108,
0.1101087779,
0.0030397107,
0.4774034321,
0.2828223109,
0.2613269091,
0.5220366716,
0.2338138521,
-0.0255850572,
-0.3653489649,
0.2001155168,
0.5069090724,
-0.3683281541,
-0.0568225831,
-0.04391516,
0.2323501855,
-0.2388921827,
-0.3795405924,
-0.2639642656,
0.3889551461,
-0.1608817875,
-0.2568490207,
0.2435088605,
-0.047355976,
0.0063970075,
0.1846164763,
-0.2035259455,
0.1530352831,
0.807575345,
0.1228646785,
-0.0009973975,
-0.3829838037,
-0.3443287313,
-0.1443083733,
0.1634519994,
-0.1493181735,
0.1704650223,
-0.3239408433,
-0.0275483225,
-0.0192408282,
0.2792572379,
0.359027952,
0.2796683609,
-0.1201950833,
0.0742158368,
-0.0097499536,
0.1716917008,
0.0075827306,
0.1788503826,
0.0395966582,
0.1739645153,
0.1511009037,
-0.1174860597,
-0.0779492259,
-0.4345598817,
0.0654477701,
0.3959385753,
-0.1649540812,
0.1745498627,
-0.3532509208,
-0.1534026265,
-0.0058773616,
0.2983110845,
-0.2523604631,
-0.0957888588,
0.5177612901,
-0.4819995165,
0.0849935487,
-0.1764228344,
0.0201749615,
-0.0753380135,
0.5530557632,
0.2912755907,
-0.0139782429,
-0.3776096106,
-0.1037474573,
-0.5993497968,
-0.0270024762,
-0.3273998201,
0.2998806536,
0.0710290745,
0.2913453877,
0.0507973433,
0.1112926528,
0.4081787765,
-0.0945438966,
-0.0640786216,
0.3450732529,
-0.3213585019,
0.4485046864,
-0.2388507724,
-0.1924461871,
-0.0728884488,
-0.4286518097,
0.3931478262,
0.069162257,
-0.150486961,
-0.1682019234,
-0.0282134209,
0.1636441499,
0.152528733,
0.1952545792,
0.3520744443,
0.3359539509,
0.0250957534,
-0.0006475982,
-0.1216745898,
0.2023513019,
-0.0488462485,
0.320684582,
-0.0580116697,
0.0556004867,
-0.2994980812,
0.1774425954,
0.1082617193,
-0.1121884808,
0.0085698813,
-0.2228359729,
-0.336959213,
-0.0309145711,
-0.0465861596,
0.3373243511,
0.0686804131,
0.3729418218,
0.1067380235,
-0.0717499703,
-0.1552369297,
-0.106937103,
-0.0228312965,
-0.288187772,
-0.3033369482,
-0.4513739645,
0.2482391447,
-0.2632822096,
0.1336895078,
-0.2243405581,
-0.3340467215,
0.3018876016,
-0.0021041867,
-0.3622485995,
0.3494460881,
-0.4049950838,
-0.0751621574,
-0.1555634141,
0.0664820448,
-0.074796766,
-0.4459966719,
0.2267223001,
-0.1301654726
]
|
https://github.com/huggingface/datasets/issues/1992 | `datasets.map` multi processing much slower than single processing | Thank you for the reply! I would be happy to follow the discussions related to the issue.
If you do not mind, could you also give a little more explanation on my p.s.2? I am having a hard time figuring out why the single processing `map` uses all of my cores.
@lhoestq @theo-m | Hi, thank you for the great library.
I've been using datasets to pretrain language models, and it often involves datasets as large as ~70G.
My data preparation step is roughly two steps: `load_dataset` which splits corpora into a table of sentences, and `map` converts a sentence into a list of integers, using a tokenizer.
I noticed that `map` function with `num_proc=mp.cpu_count() //2` takes more than 20 hours to finish the job where as `num_proc=1` gets the job done in about 5 hours. The machine I used has 40 cores, with 126G of RAM. There were no other jobs when `map` function was running.
What could be the reason? I would be happy to provide information necessary to spot the reason.
p.s. I was experiencing the imbalance issue mentioned in [here](https://github.com/huggingface/datasets/issues/610#issuecomment-705177036) when I was using multi processing.
p.s.2 When I run `map` with `num_proc=1`, I see one tqdm bar but all the cores are working. When `num_proc=20`, only 20 cores work.
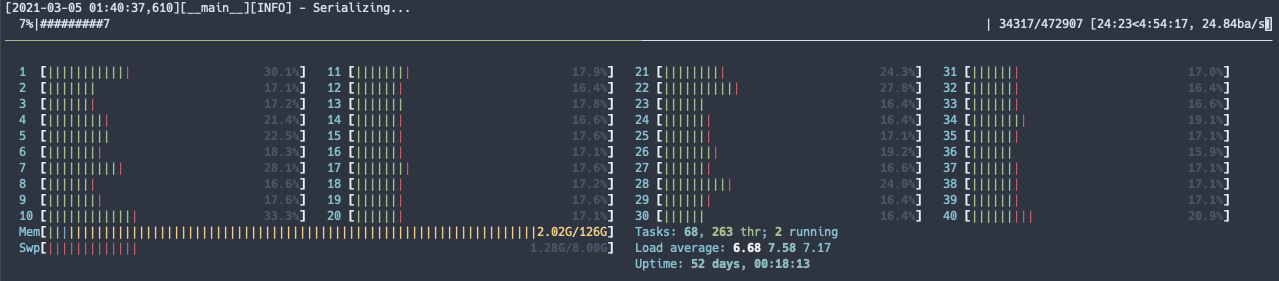
| 53 | `datasets.map` multi processing much slower than single processing
Hi, thank you for the great library.
I've been using datasets to pretrain language models, and it often involves datasets as large as ~70G.
My data preparation step is roughly two steps: `load_dataset` which splits corpora into a table of sentences, and `map` converts a sentence into a list of integers, using a tokenizer.
I noticed that `map` function with `num_proc=mp.cpu_count() //2` takes more than 20 hours to finish the job where as `num_proc=1` gets the job done in about 5 hours. The machine I used has 40 cores, with 126G of RAM. There were no other jobs when `map` function was running.
What could be the reason? I would be happy to provide information necessary to spot the reason.
p.s. I was experiencing the imbalance issue mentioned in [here](https://github.com/huggingface/datasets/issues/610#issuecomment-705177036) when I was using multi processing.
p.s.2 When I run `map` with `num_proc=1`, I see one tqdm bar but all the cores are working. When `num_proc=20`, only 20 cores work.
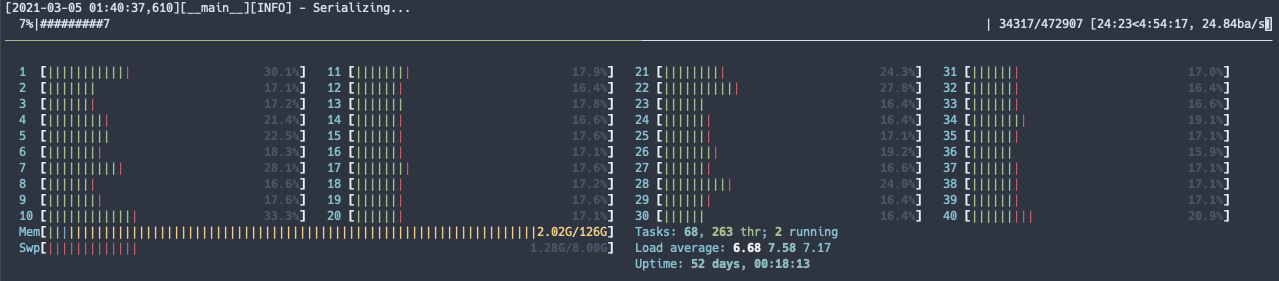
Thank you for the reply! I would be happy to follow the discussions related to the issue.
If you do not mind, could you also give a little more explanation on my p.s.2? I am having a hard time figuring out why the single processing `map` uses all of my cores.
@lhoestq @theo-m | [
-0.3987976611,
-0.3398563266,
-0.091953449,
0.3478133082,
-0.1069305539,
0.0302639306,
0.3706740737,
0.1026252583,
0.0623085685,
0.0149739897,
0.0955683589,
0.4472280145,
0.174067989,
0.198543638,
-0.1503284425,
0.032466758,
0.2079120129,
-0.0139357867,
0.208007589,
-0.0224976484,
-0.1496806145,
0.167601645,
-0.4635995328,
-0.0011215465,
-0.4276062846,
-0.1505297571,
0.1084921807,
0.158368662,
-0.1540080458,
-0.2310483158,
-0.2729550004,
0.1561858207,
-0.0772200748,
0.5987080336,
-0.0001209586,
-0.1784320474,
-0.0154783335,
0.2657154202,
0.0826333389,
0.0226128697,
-0.1489183009,
-0.2439105213,
-0.050877247,
-0.1065186933,
0.1241560504,
0.2492049038,
-0.0815335363,
-0.4744797647,
0.0348684639,
0.0918458477,
0.0700883493,
0.3698398173,
-0.3573280573,
0.0058347485,
-0.400841713,
0.1153549626,
-0.1398286521,
0.0462570339,
0.3084879518,
-0.1706032604,
-0.1413282603,
0.3538629413,
-0.05107731,
0.3019240797,
-0.0005347754,
-0.0917704403,
0.0509420261,
-0.4716904163,
0.2142588943,
0.3441272378,
0.0050044041,
-0.0449157991,
-0.1534074545,
-0.2339179069,
-0.3566296101,
-0.1425355822,
0.1976320446,
0.1191562638,
0.1468938887,
0.0236070026,
-0.5594056845,
0.1424555629,
0.3238298893,
-0.0603598654,
-0.1207490787,
0.034040872,
0.102419205,
0.324911207,
0.3525270522,
0.158886835,
-0.0753306448,
-0.2213249505,
0.3150900304,
0.1927133352,
-0.7903565764,
-0.0546726659,
0.2171827853,
-0.0919131339,
-0.0570157617,
-0.1511586159,
-0.1151764542,
0.3496940732,
-0.3474546373,
0.0873435363,
0.317974478,
-0.0674330294,
0.0333348997,
0.077857241,
-0.0398388207,
-0.1619226485,
-0.2981228828,
0.0592451803,
0.0821142122,
-0.309110254,
-0.0677428991,
0.1036741883,
-0.3128350675,
-0.0841978714,
-0.1708450913,
0.0062152678,
-0.2175656557,
-0.0867864266,
0.1496062577,
0.0932786837,
-0.0010675606,
0.8402993083,
-0.2644472718,
0.093375273,
-0.4438732862,
-0.6254181862,
-0.0214880016,
-0.0977301523,
-0.3783907592,
0.2289130986,
0.120244965,
-0.0522695147,
0.129993543,
0.3237180114,
0.0419280082,
-0.1985152364,
0.3197095692,
-0.5415102243,
0.1895831674,
0.0605314896,
0.1435219347,
0.5663230419,
-0.0963239148,
0.2923191786,
-0.1278512776,
0.2347624749,
-0.5155059695,
-0.2475563139,
0.1758902222,
-0.0223861281,
0.1043803319,
0.1009874269,
-0.4531757534,
0.5067194104,
0.2928760946,
-0.195936799,
-0.1508531421,
-0.1562155187,
-0.6555333138,
-0.1608220935,
-0.0710844398,
0.1317823827,
-0.3583335578,
0.2057324797,
-0.3523509502,
0.1483931988,
0.407055378,
0.6043969989,
-0.2138479501,
0.3782387078,
0.0245644506,
0.2347586751,
0.0038244443,
-0.0866081268,
-0.3828225732,
0.5263624191,
-0.2283225656,
-0.0003066051,
-0.1912579685,
0.1750386655,
0.2384457588,
-0.0272581354,
0.3657390773,
0.2632679939,
0.0094162375,
0.3835634589,
-0.2240214646,
-0.1533070952,
0.163163349,
0.0522792786,
-0.0803730115,
-0.1023803577,
-0.0463995747,
-0.2913084924,
0.3220102489,
-0.0063353125,
0.0290318858,
0.4283188879,
-0.3774927557,
-0.1908319592,
-0.1373766065,
-0.2449977696,
-0.0953906327,
0.3824571967,
0.0239037871,
0.070638068,
0.4634763002,
0.038680695,
0.1495972574,
0.1042517871,
-0.0175235365,
-0.0793732256,
-0.0917162374,
-0.1016783342,
-0.1805592179,
-0.2226994783,
-0.0659700185,
0.4419492483,
0.222758323,
-0.0988936052,
-0.039299462,
-0.1586316526,
0.0430583023,
0.0839976892,
-0.2125140429,
-0.0789049566,
0.0700057223,
0.0641729385,
-0.0729279369,
0.2602722943,
0.4253131747,
-0.0739463866,
0.1746498644,
0.1128145903,
0.3552787304,
-0.0458753444,
0.0203689262,
-0.1413393766,
0.1449813843,
-0.2591529489,
-0.0521304868,
0.2841013968,
0.0838300809,
0.4506698847,
0.0235610437,
-0.1425479203,
-0.0140933674,
0.2643782198,
0.1708125323,
0.0492692702,
0.3555655777,
0.3383414447,
0.234953329,
0.3360344768,
-0.1631884128,
0.3445367515,
0.6126604676,
0.0417518467,
-0.3295568824,
0.0292304493,
-0.2006421089,
-0.3668962419,
0.0839065388,
-0.1144671887,
0.5725329518,
0.0204459783,
0.2464560121,
-0.0426015742,
-0.0617182776,
-0.0788925216,
-0.0321187302,
0.1035837308,
0.2068731785,
-0.1335343122,
0.2963626087,
-0.0233535655,
-0.0464380533,
-0.2598058581,
0.3200157583,
0.216746375,
-0.2419923693,
0.130570963,
-0.3196634948,
0.1452296823,
0.2040528208,
-0.1186888441,
-0.3031509519,
-0.2074020803,
-0.1162160337,
-0.0619461648,
0.2765517533,
-0.0591163114,
0.2854480743,
-0.0924615338,
-0.1519616991,
-0.0172396973,
-0.0202097539,
-0.1655509025,
-0.1726309508,
0.0085462546,
0.2644036412,
0.2206044495,
0.0475902073,
-0.0641813204,
-0.2819518745,
-0.0524871573,
-0.1731323451,
-0.1106104329,
0.1379326433,
0.0642574653,
-0.171105355,
0.0039565582,
-0.2347947359,
0.1894124299,
0.1470808983,
-0.2577964962,
-0.1638475657,
0.0355856009,
-0.1214442626,
-0.2122677118,
0.0128886104,
-0.1658167392,
-0.0854586884,
-0.095539093,
0.3060118258,
-0.1661305428,
0.3232093453,
-0.1944456249,
0.0642023534,
-0.0389950275,
-0.0717668608,
0.03121024,
-0.345269382,
-0.3713633716,
-0.0210940521,
0.0280968081,
-0.2007674426,
-0.1226665601,
0.0851426125,
0.2075580657,
0.1145336106,
-0.1510455459,
0.1748337448,
-0.3728003204,
0.1301905215,
0.0589382201,
0.0784904435,
0.4467202127,
0.0360535122,
0.0617041327,
0.0150989797,
-0.4115450084,
-0.0789793059,
0.2864607573,
0.0288675241,
0.028507119,
0.3272490501,
0.0790636316,
0.6817414165,
0.4720705748,
-0.1880874336,
0.1948012859,
0.0043177176,
-0.1779789776,
-0.3762972653,
-0.2214649618,
0.1091213673,
-0.2775558829,
0.1900645345,
0.4069155157,
0.0025336032,
-0.4038465619,
-0.0298231412,
0.2467473298,
-0.2389830947,
-0.0058508487,
0.1471873969,
-0.0795925632,
0.128286019,
0.2544083595,
-0.1898123175,
-0.3439294994,
-0.0338944197,
-0.0574087501,
-0.0707048625,
-0.1244393513,
-0.2597435713,
-0.5114725828,
-0.0330196992,
-0.3813464642,
0.2475262433,
0.0228804834,
0.2694842815,
-0.0378904268,
0.1243411079,
0.3278180063,
-0.0064776321,
0.531437695,
-0.373039633,
-0.172452122,
0.1234859079,
-0.4129563868,
-0.2700181603,
0.1126654446,
-0.1809011549,
0.2845950127,
0.5743812323,
0.5144298673,
-0.0455429181,
-0.2244945318,
-0.0374760479,
0.1309110373,
0.1329892278,
-0.2703754306,
-0.3282872736,
0.1430772543,
-0.1507305205,
0.1006361619,
-0.0207588878,
0.136767,
0.1283371001,
-0.1023594216,
0.0580425896,
0.0914532021,
0.2539270818,
0.1980282813,
0.096308127,
0.0602106377,
0.2583251894,
0.2657181025,
-0.0980603993,
0.0382844098,
0.3328792751,
-0.154834941,
-0.0922506452,
0.2330234647,
0.0603710748,
0.3217385709,
0.3481731117,
0.1211911812,
0.0601414889,
0.1878472865,
0.2428903729,
-0.2255193442,
0.2873826623,
0.3583305478,
0.368776381,
-0.4462332129,
-0.4971280396,
-0.0129834199,
0.4985201657,
-0.1683436185,
0.3029262424,
-0.7764914632,
0.0737234652,
0.0215334632,
0.0744742453,
0.7982974052,
-0.4352928996,
0.062213812,
-0.2951286733,
0.2099919021,
-0.0567075163,
-0.596722424,
0.1344831735,
-0.1911982,
-0.3782000244,
0.0069170073,
-0.1047016829,
0.1857599169,
0.5643202662,
0.1523875892,
0.2857615352,
0.3366233706,
0.0805623233,
-0.0095908847,
0.3668849766,
0.5028188825,
-0.3357383311,
0.154033795,
0.0463158637,
0.1937488914,
-0.1932335645,
0.0020393606,
0.0996448919,
-0.071917586,
-0.0027786617,
-0.314311713,
-0.218389824,
-0.4510263503,
0.2037435919,
-0.0680122972,
0.3175692558,
0.3931637108,
0.2769824862,
-0.0670499802,
0.0411295034,
0.0065711495,
0.0356716365,
0.3010513186,
0.2570821345,
0.3631036878,
-0.4733555019,
-0.0057051382,
-0.1186289936,
-0.2454584241,
-0.2174408585,
-0.1700526327,
-0.0586602017,
-0.1510438323,
0.487613678,
0.157854259,
0.1609753817,
-0.190501824,
0.2473521233,
0.1159882769,
-0.1148681939,
-0.0019466252,
0.1373943835,
0.0247034431,
0.6386432052,
-0.2702285349,
-0.4856572449,
-0.0345761888,
0.3869276047,
0.2485568374,
-0.1072566286,
0.0116948104,
0.2025441378,
-0.2665694952,
-0.1496109217,
0.1637886316,
-0.0857771188,
-0.072998777,
0.1273658276,
0.0348298512,
-0.249417156,
0.2480976135,
-0.100148268,
-0.1314287186,
-0.0336117595,
-0.2042175829,
-0.2643252015,
-0.2544718087,
-0.1720086485,
-0.3750602305,
-0.2070090324,
0.2421012223,
-0.0172376316,
0.1284519285,
0.469553709,
-0.1497865021,
0.0607125647,
0.1021557823,
0.1906089932,
-0.0384998247,
-0.2601142228,
0.094197996,
-0.0170743745,
-0.0643606856,
0.0783961266,
-0.085348092,
-0.1668204367,
-0.0115813902,
0.1847727001,
-0.0130351288,
-0.2575169802,
0.3359078765,
-0.1025238931,
-0.1739263088,
-0.3928407431,
-0.0501776151,
0.0468401089,
0.0097015193,
-0.1023312882,
0.317299366,
-0.2931719124,
-0.3031932414,
0.4068656862,
-0.2091908455,
-0.0665454566,
-0.0005235064,
0.2697985172,
0.4595625699,
-0.0918449834,
-0.1277806014,
0.0299207419,
0.2942762971,
0.0688003972,
0.1120860726,
-0.1015249416,
0.1829240024,
0.2699060738,
0.2781373262,
0.1202000529,
0.1141074821,
-0.2037567049,
0.0053947573,
0.0241429377,
-0.2130513191,
-0.1793759316,
0.5706431866,
0.0138294073,
0.1518812478,
0.068956241,
0.321791321,
0.2287773192,
-0.001245395,
-0.1071083546,
0.0849716067,
-0.3123014569,
0.0684469864,
0.1110092551,
0.2458713502,
0.2573177516,
0.4362217784,
0.1256208867,
0.0101208827,
0.4173810482,
0.2422874123,
0.3094067574,
0.5200666785,
0.2401480973,
0.0135989767,
-0.3639060259,
0.1917427927,
0.4900938272,
-0.3441902101,
-0.0662107319,
-0.0487065464,
0.2394540906,
-0.2684267759,
-0.3709074259,
-0.2196555436,
0.390693754,
-0.1724592596,
-0.2432680726,
0.2041934878,
-0.0381440707,
-0.0459647514,
0.1604243666,
-0.2366207391,
0.1313252449,
0.8348479271,
0.1233758554,
0.0309464894,
-0.3607611358,
-0.3917116821,
-0.1342183799,
0.1480795592,
-0.1345746219,
0.1902973503,
-0.3134203851,
-0.0068785818,
-0.0136600668,
0.276581347,
0.3565855026,
0.2774434388,
-0.1138226837,
0.0915467441,
-0.0268792175,
0.153975293,
-0.0184285436,
0.1534866542,
0.0066651679,
0.1805171371,
0.1535552889,
-0.0864600316,
-0.098198764,
-0.4284716845,
0.0447323285,
0.339898169,
-0.1634694636,
0.1326927841,
-0.3101339042,
-0.1590602547,
-0.0120578529,
0.2858177423,
-0.2495388687,
-0.0862629265,
0.4984058142,
-0.490280509,
0.0726508498,
-0.1841206998,
0.0356181376,
-0.0405582972,
0.5247300863,
0.2865369022,
-0.0129879164,
-0.4199316502,
-0.1138789356,
-0.5841238499,
-0.0655892715,
-0.3243658841,
0.2615007758,
0.0450748056,
0.2684962451,
0.0611372516,
0.1447687745,
0.4047617614,
-0.1011889651,
-0.0866834894,
0.3594870269,
-0.3306727707,
0.5003920197,
-0.2142935991,
-0.18564789,
-0.0553077571,
-0.4004402757,
0.3668000996,
0.0968989432,
-0.1297347099,
-0.1715719551,
-0.0162613336,
0.1787752509,
0.1407822073,
0.1806529015,
0.3315010965,
0.3267032504,
0.0269237794,
-0.0089407964,
-0.101282984,
0.219316721,
-0.079232648,
0.314540565,
-0.0376671739,
0.0590491183,
-0.301656425,
0.1776496172,
0.1021891609,
-0.1452149749,
0.0031904776,
-0.2515271604,
-0.3215588629,
-0.0398882553,
-0.0551039129,
0.3557629883,
0.089744091,
0.4125424027,
0.1387647986,
-0.0893785655,
-0.2004951835,
-0.0998042524,
-0.0184922963,
-0.2824785411,
-0.323176682,
-0.446031183,
0.2157074958,
-0.2852637768,
0.1410281807,
-0.2329759151,
-0.3740325868,
0.3034422994,
-0.0229324419,
-0.4084105194,
0.3051840067,
-0.3485662341,
-0.0823977664,
-0.1502870172,
0.0432495698,
-0.0781465173,
-0.4487423003,
0.2001757026,
-0.1336034089
]
|
https://github.com/huggingface/datasets/issues/1992 | `datasets.map` multi processing much slower than single processing | Regarding your ps2: It depends what function you pass to `map`.
For example, fast tokenizers from `transformers` in Rust tokenize texts and parallelize the tokenization over all the cores. | Hi, thank you for the great library.
I've been using datasets to pretrain language models, and it often involves datasets as large as ~70G.
My data preparation step is roughly two steps: `load_dataset` which splits corpora into a table of sentences, and `map` converts a sentence into a list of integers, using a tokenizer.
I noticed that `map` function with `num_proc=mp.cpu_count() //2` takes more than 20 hours to finish the job where as `num_proc=1` gets the job done in about 5 hours. The machine I used has 40 cores, with 126G of RAM. There were no other jobs when `map` function was running.
What could be the reason? I would be happy to provide information necessary to spot the reason.
p.s. I was experiencing the imbalance issue mentioned in [here](https://github.com/huggingface/datasets/issues/610#issuecomment-705177036) when I was using multi processing.
p.s.2 When I run `map` with `num_proc=1`, I see one tqdm bar but all the cores are working. When `num_proc=20`, only 20 cores work.
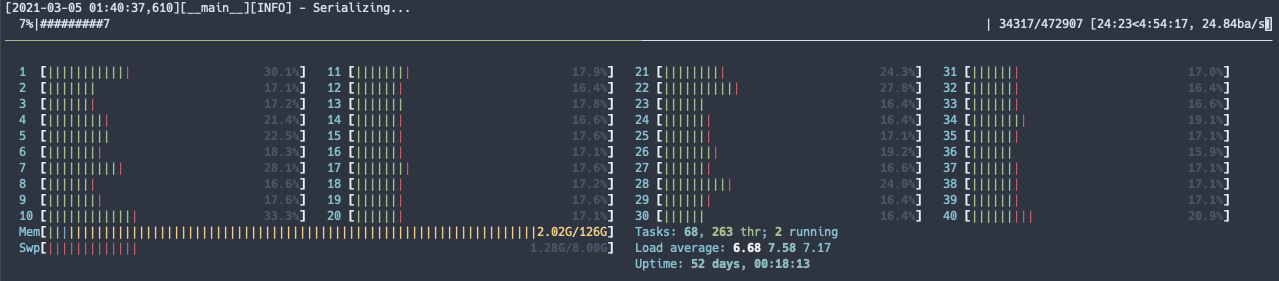
| 29 | `datasets.map` multi processing much slower than single processing
Hi, thank you for the great library.
I've been using datasets to pretrain language models, and it often involves datasets as large as ~70G.
My data preparation step is roughly two steps: `load_dataset` which splits corpora into a table of sentences, and `map` converts a sentence into a list of integers, using a tokenizer.
I noticed that `map` function with `num_proc=mp.cpu_count() //2` takes more than 20 hours to finish the job where as `num_proc=1` gets the job done in about 5 hours. The machine I used has 40 cores, with 126G of RAM. There were no other jobs when `map` function was running.
What could be the reason? I would be happy to provide information necessary to spot the reason.
p.s. I was experiencing the imbalance issue mentioned in [here](https://github.com/huggingface/datasets/issues/610#issuecomment-705177036) when I was using multi processing.
p.s.2 When I run `map` with `num_proc=1`, I see one tqdm bar but all the cores are working. When `num_proc=20`, only 20 cores work.
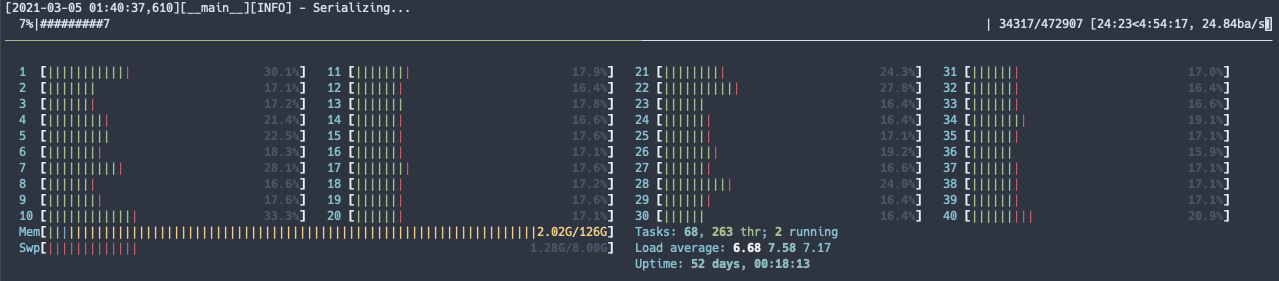
Regarding your ps2: It depends what function you pass to `map`.
For example, fast tokenizers from `transformers` in Rust tokenize texts and parallelize the tokenization over all the cores. | [
-0.4439505339,
-0.2767179608,
-0.0788298398,
0.375110507,
-0.0908034071,
-0.005079166,
0.3211758435,
0.0643403158,
-0.0458023325,
-0.009251467,
0.0477654599,
0.4086365104,
0.2179488242,
0.1524447352,
-0.14575167,
-0.001972422,
0.2254884541,
-0.021348143,
0.1467745155,
0.0173892658,
-0.2218794972,
0.102797471,
-0.5076212287,
0.0070181177,
-0.4328961074,
-0.1630928963,
0.0852131322,
0.0920832679,
-0.1628725082,
-0.2309197932,
-0.2925642133,
0.209413901,
-0.0740562826,
0.5870193839,
-0.0001180967,
-0.1688384414,
-0.0100618778,
0.2632312477,
0.1063560694,
0.0013799567,
-0.0580673292,
-0.2970839143,
-0.0959244668,
-0.0974918082,
0.1089046672,
0.1052419096,
-0.0769349635,
-0.4238999784,
0.1259803772,
0.0137933996,
0.1001286805,
0.3818843365,
-0.2749177516,
-0.0349338278,
-0.3510385454,
0.1526193023,
-0.1374157667,
-0.024036942,
0.2417444289,
-0.124066405,
-0.127266705,
0.3543686867,
-0.1285182536,
0.2587578893,
0.0789576247,
-0.0478512757,
0.0509214364,
-0.457182169,
0.1748204082,
0.4000488818,
0.012555548,
-0.0977234617,
-0.1687846631,
-0.2309558988,
-0.279165864,
-0.1573260576,
0.2020866871,
0.1040828675,
0.1339536905,
-0.0070666522,
-0.6016132236,
0.2399574369,
0.3542023599,
-0.1202203333,
-0.116099216,
0.0890452638,
0.1198708564,
0.3441288173,
0.3367705941,
0.0770299509,
-0.0875309408,
-0.2567175031,
0.2177377343,
0.2279638648,
-0.8232133389,
-0.0756710246,
0.221240893,
-0.167631641,
-0.0521671847,
-0.1305062026,
-0.1465458125,
0.356933713,
-0.3285426199,
0.119299531,
0.2981947064,
-0.0345785767,
0.0140543319,
0.1581132412,
-0.0102360221,
-0.2339848727,
-0.3126997054,
0.0593382306,
0.0346815176,
-0.2625655234,
-0.0355954506,
0.0848607868,
-0.3532975614,
-0.0575249717,
-0.2106845081,
0.0534193404,
-0.2790290415,
-0.0660478547,
0.1619873792,
0.1405693144,
0.0106095644,
0.7866416574,
-0.3018145561,
0.0486738347,
-0.3648938835,
-0.5528380871,
-0.0429502912,
-0.1930679381,
-0.3997561932,
0.2632678151,
0.1055118591,
0.0493487529,
0.1494627744,
0.2821130455,
0.0765935928,
-0.1413560808,
0.3494643867,
-0.5429765582,
0.1496422589,
0.0726191998,
0.1545604318,
0.517794013,
-0.1384747475,
0.314902097,
-0.1971449852,
0.2203018814,
-0.4752670527,
-0.2318788171,
0.215132758,
0.013539305,
0.0885787606,
0.0796748102,
-0.5455653071,
0.5509294868,
0.2919280231,
-0.1726648808,
-0.1275852323,
-0.1853659451,
-0.6103125811,
-0.1781905591,
-0.001849053,
0.1226011813,
-0.2417798787,
0.1732542813,
-0.2710023522,
0.1222161204,
0.4497341216,
0.6742505431,
-0.2196298838,
0.424621582,
0.015841281,
0.3035618663,
0.0449150912,
-0.1139075756,
-0.3109883964,
0.4910984635,
-0.1610905677,
-0.0346944816,
-0.181156069,
0.1404017359,
0.3190975189,
-0.0290231537,
0.2742300332,
0.3147365749,
0.0265183449,
0.3793387413,
-0.1575172693,
-0.1305786818,
0.1962331682,
0.0451969318,
-0.115042977,
-0.135610044,
-0.0503522716,
-0.2750129104,
0.2248006314,
-0.0303577166,
0.0487306006,
0.3911473155,
-0.3012830317,
-0.17582196,
-0.1415570527,
-0.227732867,
-0.0599742495,
0.3407978415,
0.0430903286,
0.0639525726,
0.4608147144,
0.0015958071,
0.2276875377,
0.1355304718,
-0.0380947143,
-0.1376335621,
-0.0313481614,
-0.0491698943,
-0.1346634775,
-0.2262031436,
-0.0678616092,
0.3672983944,
0.2238069922,
-0.0643267706,
0.0883314386,
-0.1257751286,
0.0670795292,
0.0064628371,
-0.2424024791,
-0.0341824107,
0.0654882565,
0.1089309305,
-0.0454827882,
0.2792192996,
0.4492980242,
-0.0380162001,
0.1182728708,
0.1675734967,
0.3643092513,
-0.0421774201,
0.0124648977,
-0.127992779,
0.0809549317,
-0.2292950898,
-0.0607797876,
0.2941325307,
0.1038634256,
0.4727626741,
0.0048597846,
-0.1762063205,
0.0205459371,
0.2855570316,
0.1121590361,
0.1042971388,
0.2605011165,
0.3831949532,
0.292907387,
0.380749166,
-0.1670196503,
0.2910592854,
0.5920897722,
-0.0072924332,
-0.3589829504,
0.0816679224,
-0.2233638614,
-0.4092293978,
0.0779986084,
-0.1123960018,
0.5411707759,
0.0466135889,
0.2133835405,
-0.0555461869,
-0.1274145544,
-0.0524572618,
-0.0064761201,
0.0726006925,
0.197327897,
-0.0815939382,
0.2709233165,
0.0563163459,
-0.0644894093,
-0.2889433801,
0.3361005783,
0.2415704429,
-0.2043417096,
0.076001294,
-0.2641550601,
0.1124652922,
0.1709751189,
-0.060718134,
-0.2124260217,
-0.1904429644,
-0.1214683205,
-0.1239399016,
0.1627361476,
-0.0067013903,
0.2737025023,
-0.0764564723,
-0.0927326828,
-0.0275583062,
-0.0323879868,
-0.1445225179,
-0.1797597557,
0.0104284203,
0.2918091714,
0.2076406479,
0.1169968694,
-0.0755056217,
-0.2324869633,
-0.0665044636,
-0.2315774262,
-0.0556142479,
0.0795417801,
0.0135090183,
-0.1537782103,
-0.0750606731,
-0.2716275752,
0.046104718,
0.19815965,
-0.3055954576,
-0.2410658747,
0.0426127836,
-0.1434979439,
-0.2572675049,
0.0451564789,
-0.1229170635,
-0.0881871432,
-0.108315669,
0.3268549442,
-0.1563324779,
0.2969707847,
-0.2059315294,
0.042374149,
-0.0350833423,
-0.1536308676,
0.0389461964,
-0.3043985665,
-0.3341439366,
0.0511072874,
-0.0429351702,
-0.1612560004,
-0.1311006844,
0.1183315888,
0.1708221585,
0.1495732665,
-0.1346122622,
0.1372233629,
-0.3764241934,
0.1445038021,
0.0494961403,
0.1159210429,
0.4347305298,
0.0688750595,
0.0020962104,
0.0667256191,
-0.4192671478,
-0.0635161176,
0.2809039354,
0.051003091,
0.0156696867,
0.390145123,
0.0600491576,
0.6509932876,
0.5063644648,
-0.1862696409,
0.1578830779,
0.0148957409,
-0.1145069972,
-0.4058782458,
-0.170289278,
0.1095638573,
-0.2204570323,
0.1181805134,
0.4101577699,
-0.0052145692,
-0.4526441693,
0.0020454535,
0.2613651156,
-0.2650692165,
-0.0189335663,
0.1734434664,
-0.10557919,
0.1956782788,
0.1890767068,
-0.1457656324,
-0.3188576698,
-0.0576308966,
-0.0498396568,
-0.1312031597,
-0.1142139062,
-0.2327938676,
-0.5820651054,
0.0001043196,
-0.3677586317,
0.2439449877,
0.0063361935,
0.2492843717,
0.0271377917,
0.0890654474,
0.327380389,
-0.0116159609,
0.4494199455,
-0.3170933723,
-0.191744104,
0.1078536063,
-0.4520252943,
-0.2145801634,
0.0766328201,
-0.1998128593,
0.2188529074,
0.607011497,
0.5290970206,
-0.1593934894,
-0.242448777,
-0.0145312762,
0.067507349,
0.2063866705,
-0.2496497929,
-0.2906809449,
0.1218741089,
-0.1460336596,
0.1630270034,
-0.0644272789,
0.1508836299,
0.0983757675,
-0.1814579368,
0.0365006961,
0.0545810685,
0.2548567653,
0.2476996779,
0.1457829922,
0.0650622323,
0.3104208112,
0.3232927024,
-0.1338670999,
0.1037749574,
0.2178994715,
-0.0925103351,
-0.1132436767,
0.1909928173,
0.0520566702,
0.3045854867,
0.3385305703,
0.0856507123,
0.0982143283,
0.0943280458,
0.3066306412,
-0.2347386926,
0.3093482852,
0.4002846777,
0.4037313461,
-0.4667280316,
-0.5559287667,
-0.0690210164,
0.4330457151,
-0.1820666492,
0.2211445421,
-0.7285044193,
0.0773609132,
0.1097152904,
0.1712524742,
0.8330785036,
-0.4548244774,
0.0848215595,
-0.3236432076,
0.2537368536,
0.0580801442,
-0.7181648016,
0.0962229371,
-0.2373635918,
-0.2546756268,
0.0246202443,
-0.1100669727,
0.1980310231,
0.5148919821,
0.1154823899,
0.2528630495,
0.4243819714,
0.2372803688,
-0.0995558575,
0.3643095791,
0.4965113997,
-0.4183228612,
0.1333129406,
0.0802546367,
0.1976225674,
-0.2389123738,
0.0270953644,
0.0834895447,
-0.0795703903,
0.0184417851,
-0.3758704364,
-0.2915438116,
-0.4238472581,
0.2038733959,
-0.0037292533,
0.2687774599,
0.3288629949,
0.2797587514,
-0.0635960996,
0.1441979855,
-0.0059175771,
0.0863513499,
0.2842146158,
0.2989467382,
0.3509504795,
-0.5309545398,
-0.0671194047,
-0.0798983201,
-0.2635247409,
-0.1854158491,
-0.1425006539,
-0.1600114554,
-0.1232454181,
0.3970692456,
0.1707145721,
0.1685150266,
-0.1710098982,
0.2841367424,
0.0973165333,
-0.1147538424,
0.0305127297,
0.102427721,
-0.0632516518,
0.6600754261,
-0.2263179272,
-0.4700721204,
-0.0673077032,
0.30551669,
0.2026192993,
-0.1621506661,
0.051447317,
0.1235845983,
-0.265448153,
-0.1657326221,
0.2679198384,
-0.0332037285,
-0.063268207,
0.0777311847,
-0.0185653009,
-0.2477259785,
0.2914330363,
0.015789466,
-0.0839615539,
-0.0706938729,
-0.1701772958,
-0.2372148186,
-0.2801139057,
-0.1467527896,
-0.2820577025,
-0.15562962,
0.220224753,
0.071150668,
0.0766154155,
0.4940489829,
-0.1891955435,
-0.0150636695,
-0.0130068297,
0.2362149358,
-0.0515914336,
-0.2214391679,
0.0374454409,
-0.0237097703,
-0.027768258,
0.099092178,
-0.1186020374,
-0.1766848862,
-0.0388227701,
0.171794802,
-0.0014158557,
-0.3216921091,
0.2939104438,
-0.1387358159,
-0.236267671,
-0.4579727948,
-0.0253310949,
0.0440420397,
-0.045714803,
-0.165232718,
0.3154084682,
-0.2161611617,
-0.276258111,
0.410930723,
-0.1830656826,
-0.0780496299,
0.0185389314,
0.2842988074,
0.4484981894,
-0.0958242491,
-0.0226855054,
0.0760350674,
0.3730261922,
0.0505047627,
0.1415213197,
-0.0844872743,
0.1599354446,
0.2427991182,
0.3568879068,
0.1891922206,
0.1293493658,
-0.280151397,
0.0930409878,
0.0573858321,
-0.1989367306,
-0.1231897175,
0.490380466,
0.0182052851,
0.1448255777,
0.0927429125,
0.3649457097,
0.3037866652,
0.0161977094,
-0.1273454279,
0.1338042915,
-0.2889989018,
0.072449185,
0.0860037804,
0.1859660894,
0.2598798871,
0.4262657762,
0.0697907731,
0.0363900848,
0.4503246844,
0.2709547281,
0.3376747668,
0.4775070548,
0.3344378173,
-0.0255361479,
-0.3478302658,
0.2448359877,
0.4501990378,
-0.3315545321,
-0.1329410225,
-0.0553731471,
0.1937810034,
-0.2377571762,
-0.3416782916,
-0.2749474347,
0.4440901279,
-0.2080301344,
-0.3045455813,
0.1787306368,
-0.0995023698,
-0.0396525413,
0.198478505,
-0.2173382044,
0.1424005628,
0.7284571528,
0.0734273642,
0.0488698035,
-0.390539825,
-0.2922238111,
-0.1570208967,
0.1194630712,
-0.1403241307,
0.2661328018,
-0.2927121818,
0.0441740267,
0.0352611206,
0.3550225496,
0.3926253319,
0.3303476274,
-0.1617080867,
0.0841673985,
-0.0571858399,
0.1578367054,
-0.0234030616,
0.2517148256,
0.021942528,
0.0856103376,
0.1510847956,
-0.0631430596,
-0.1325366944,
-0.501190424,
-0.0569898933,
0.2782663703,
-0.1450001299,
0.2023574263,
-0.2890168428,
-0.1213660017,
0.0647264495,
0.3031776547,
-0.2443996966,
-0.0538227595,
0.4503953159,
-0.4847293198,
0.0268956441,
-0.1731548607,
0.0600578077,
-0.0299802739,
0.5599965453,
0.2798053026,
-0.0255499799,
-0.4537345171,
-0.1623741984,
-0.6362351179,
-0.0669204295,
-0.2945362628,
0.2655956149,
0.0150778368,
0.29245013,
0.0856205449,
0.22426413,
0.3306144774,
0.0055515757,
-0.1317664683,
0.3757701814,
-0.2399875969,
0.5442320704,
-0.2748651803,
-0.1883131713,
-0.0854739025,
-0.4263991117,
0.3769554198,
0.1100715995,
-0.1097063646,
-0.2021089792,
0.0195114538,
0.2056245655,
0.1438641697,
0.2136922777,
0.3713987172,
0.3717585206,
0.0488743484,
0.0633164123,
-0.1134326309,
0.1278330088,
-0.0979391783,
0.2556671202,
-0.1105474606,
0.0603191331,
-0.2797143459,
0.1180017814,
0.0593304187,
-0.1554955244,
-0.0002601195,
-0.2810497284,
-0.3650559485,
-0.0507836007,
-0.1279811412,
0.274059236,
0.0406715684,
0.359872967,
0.1757292747,
-0.1003123447,
-0.2375797033,
-0.0797622949,
-0.020334376,
-0.3013189137,
-0.2677735984,
-0.5111198425,
0.1080260798,
-0.1718795896,
0.1276900172,
-0.2887655199,
-0.3083531857,
0.3282151818,
-0.0181477405,
-0.4100755453,
0.3328618407,
-0.3763071597,
-0.1390820444,
-0.1773984432,
0.0250443257,
-0.1261179149,
-0.4447459877,
0.1478432864,
-0.0966655165
]
|
https://github.com/huggingface/datasets/issues/1992 | `datasets.map` multi processing much slower than single processing | I am still experiencing this issue with datasets 1.9.0..
Has there been a further investigation?
<img width="442" alt="image" src="https://user-images.githubusercontent.com/29157715/126143387-8b5ddca2-a896-4e18-abf7-4fbf62a48b41.png">
| Hi, thank you for the great library.
I've been using datasets to pretrain language models, and it often involves datasets as large as ~70G.
My data preparation step is roughly two steps: `load_dataset` which splits corpora into a table of sentences, and `map` converts a sentence into a list of integers, using a tokenizer.
I noticed that `map` function with `num_proc=mp.cpu_count() //2` takes more than 20 hours to finish the job where as `num_proc=1` gets the job done in about 5 hours. The machine I used has 40 cores, with 126G of RAM. There were no other jobs when `map` function was running.
What could be the reason? I would be happy to provide information necessary to spot the reason.
p.s. I was experiencing the imbalance issue mentioned in [here](https://github.com/huggingface/datasets/issues/610#issuecomment-705177036) when I was using multi processing.
p.s.2 When I run `map` with `num_proc=1`, I see one tqdm bar but all the cores are working. When `num_proc=20`, only 20 cores work.
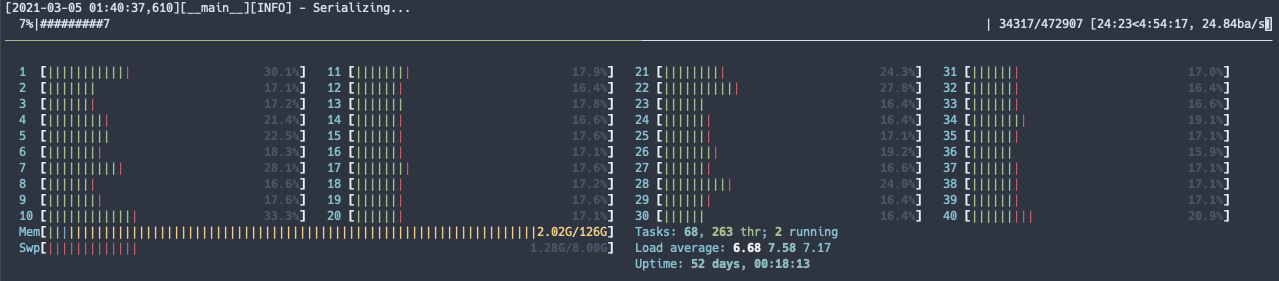
| 19 | `datasets.map` multi processing much slower than single processing
Hi, thank you for the great library.
I've been using datasets to pretrain language models, and it often involves datasets as large as ~70G.
My data preparation step is roughly two steps: `load_dataset` which splits corpora into a table of sentences, and `map` converts a sentence into a list of integers, using a tokenizer.
I noticed that `map` function with `num_proc=mp.cpu_count() //2` takes more than 20 hours to finish the job where as `num_proc=1` gets the job done in about 5 hours. The machine I used has 40 cores, with 126G of RAM. There were no other jobs when `map` function was running.
What could be the reason? I would be happy to provide information necessary to spot the reason.
p.s. I was experiencing the imbalance issue mentioned in [here](https://github.com/huggingface/datasets/issues/610#issuecomment-705177036) when I was using multi processing.
p.s.2 When I run `map` with `num_proc=1`, I see one tqdm bar but all the cores are working. When `num_proc=20`, only 20 cores work.
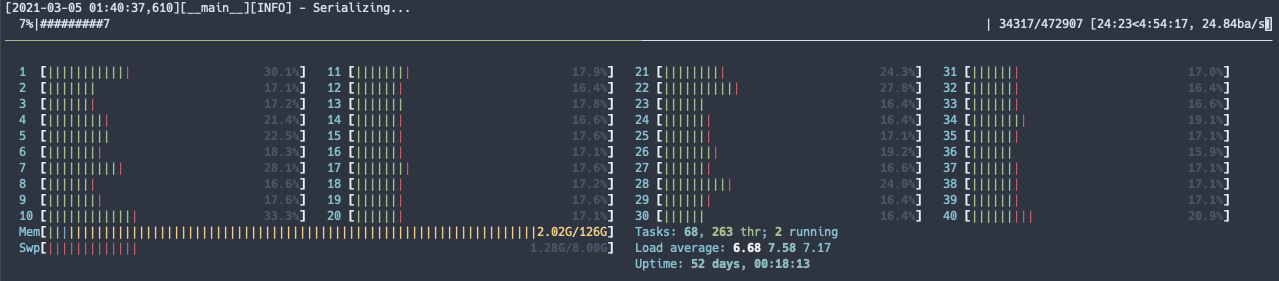
I am still experiencing this issue with datasets 1.9.0..
Has there been a further investigation?
<img width="442" alt="image" src="https://user-images.githubusercontent.com/29157715/126143387-8b5ddca2-a896-4e18-abf7-4fbf62a48b41.png">
| [
-0.4408695102,
-0.2572330236,
-0.1001467705,
0.3424951732,
-0.1136197969,
0.0254861545,
0.3359663785,
0.1372741461,
0.0238075703,
-0.0266040042,
0.072843045,
0.4267615378,
0.1649509519,
0.1844826192,
-0.1783976257,
0.0735182539,
0.1910468191,
-0.0237317272,
0.1997008175,
-0.01755937,
-0.1721213162,
0.1267191172,
-0.4863221645,
-0.0251147095,
-0.4187076688,
-0.155189544,
0.1128486544,
0.1078349277,
-0.161344856,
-0.2424039841,
-0.225424245,
0.1812608689,
-0.0989793465,
0.5712870955,
-0.0001213278,
-0.1757615209,
0.0262494031,
0.2655773759,
0.0566575825,
0.0632180572,
-0.1749771535,
-0.2656293511,
-0.060312137,
-0.1064621583,
0.1302662343,
0.1959710121,
-0.0412266776,
-0.4592749178,
0.0279737711,
0.095620729,
0.0683357194,
0.3693207502,
-0.3358561993,
0.0190169215,
-0.3772294521,
0.175735876,
-0.1743130982,
0.0439391024,
0.2703152895,
-0.1895018071,
-0.1327501684,
0.2942249477,
-0.0594553761,
0.2955109477,
-0.0293099321,
-0.0800077692,
0.0435421839,
-0.4581357837,
0.2109427154,
0.3413070142,
0.0336229689,
-0.0598062389,
-0.2014715523,
-0.2505910099,
-0.313510865,
-0.0960752591,
0.188150093,
0.1586237848,
0.143742457,
0.0002748422,
-0.5647352338,
0.1250047833,
0.3329136074,
-0.0845781416,
-0.160668999,
0.0009776424,
0.1214761809,
0.3006645441,
0.3285795748,
0.1026582941,
-0.0240334291,
-0.2341751158,
0.318665415,
0.2144549936,
-0.7959992886,
-0.0313888378,
0.2321539521,
-0.1166578233,
-0.0702338442,
-0.2011481375,
-0.0824340656,
0.3807694316,
-0.3303594291,
0.0957022458,
0.3113456964,
-0.081692405,
0.0368811749,
0.1326556653,
-0.0200128686,
-0.1649897695,
-0.3204188347,
0.0855833888,
0.0375062414,
-0.3145130575,
-0.058868859,
0.1090916842,
-0.2920224965,
-0.0776978955,
-0.1529202312,
0.0577998981,
-0.2044585943,
-0.0747551918,
0.1519746631,
0.0931523815,
-0.0251010228,
0.7979211807,
-0.2888889909,
0.0677871108,
-0.4647259712,
-0.5510894656,
-0.0266885385,
-0.1435278803,
-0.3716951609,
0.2059826106,
0.1467279941,
-0.0441311672,
0.1381736845,
0.2995371521,
0.0675619468,
-0.1942553073,
0.329844445,
-0.5215238929,
0.1908667684,
0.0897700787,
0.1518648565,
0.5952267647,
-0.1308273971,
0.3086301088,
-0.1146123931,
0.2425245643,
-0.511967957,
-0.2261331826,
0.1801619381,
-0.0164729021,
0.0661157295,
0.1085791811,
-0.450710535,
0.4928019047,
0.3107572198,
-0.2005256563,
-0.1917559206,
-0.2191667855,
-0.6590132117,
-0.1549584717,
-0.0378511921,
0.1781468838,
-0.3581595719,
0.2357655764,
-0.3264366388,
0.1465032101,
0.4354631305,
0.5881886482,
-0.1908947229,
0.3742274046,
-0.016753329,
0.2168681771,
-0.0051938337,
-0.061244078,
-0.4090889692,
0.5504704714,
-0.157384336,
-0.0077368007,
-0.1996926963,
0.1322534829,
0.2520761192,
-0.0667858943,
0.2841031253,
0.2935760617,
0.0093999207,
0.3959369063,
-0.2198730856,
-0.1206629053,
0.1546422839,
0.0758328736,
-0.0520671532,
-0.1174089387,
-0.0040198504,
-0.2566075325,
0.3107917905,
0.0013410316,
0.0485638715,
0.4202735424,
-0.376527369,
-0.1814431846,
-0.1402573436,
-0.2091577798,
-0.0894148797,
0.3687588573,
-0.0112052364,
0.0369151384,
0.3978398442,
0.0250366963,
0.1763716787,
0.127601102,
-0.059195593,
-0.0834362209,
-0.0933098122,
-0.0704991296,
-0.1872858852,
-0.1743259728,
-0.0221259892,
0.4432822466,
0.2146654427,
-0.1109526306,
-0.0165356621,
-0.1274925023,
0.0751290843,
0.0695861131,
-0.1862870753,
-0.080008246,
0.0683451295,
0.0442723967,
-0.0770369098,
0.2573573887,
0.4579809904,
-0.0575391278,
0.1573873907,
0.0783575922,
0.3972079754,
-0.0391154662,
0.0224035177,
-0.1433431357,
0.1175411567,
-0.2493546903,
-0.0373166949,
0.3127609491,
0.1098828688,
0.4193719327,
0.0704617724,
-0.1443877071,
0.0071857478,
0.3014127314,
0.1643479615,
0.015056042,
0.3293915987,
0.3466157317,
0.2566783726,
0.3388877213,
-0.1154528782,
0.3463785648,
0.6238068938,
0.0678069666,
-0.3361597359,
0.0286231562,
-0.1701203585,
-0.3801423609,
0.0395856537,
-0.1131447777,
0.526943326,
0.0170898698,
0.2577414811,
-0.0255263448,
-0.11627765,
-0.0751270875,
-0.0387659632,
0.1148214638,
0.2368962467,
-0.0769186988,
0.3169466555,
-0.0354398191,
-0.0635434315,
-0.264798671,
0.3754662275,
0.2200480402,
-0.2382180989,
0.0989620537,
-0.3365549445,
0.1643038392,
0.1856618822,
-0.1233558729,
-0.2650385499,
-0.2415281236,
-0.1381920874,
-0.0913196728,
0.2174678296,
-0.0295522492,
0.2439493835,
-0.1340826601,
-0.0943094641,
-0.0267704017,
0.0108898506,
-0.1664040387,
-0.1559699476,
0.004940846,
0.2704434395,
0.2264304757,
0.0572019406,
-0.055270832,
-0.2538317442,
-0.0788091868,
-0.2205042094,
-0.0990529433,
0.1591481715,
0.0593892448,
-0.1872386932,
-0.0787216723,
-0.2335621715,
0.1749193817,
0.1698776037,
-0.2436435968,
-0.1829373986,
0.0668815002,
-0.1159513593,
-0.2466945648,
0.0244953297,
-0.1498459727,
-0.0929287821,
-0.1155195534,
0.2580093741,
-0.1713647842,
0.3255140781,
-0.1932543963,
0.0785439685,
-0.0488126799,
-0.1118041724,
-0.0171387549,
-0.3181793988,
-0.3501659036,
0.0268695783,
-0.0320225544,
-0.2036222368,
-0.1449835002,
0.067317158,
0.1803426296,
0.1953359246,
-0.1574310213,
0.1874528229,
-0.4001611471,
0.1639228612,
0.0731302723,
0.0248604771,
0.4335304201,
0.0243837591,
0.0598814562,
-0.0029196718,
-0.4167128205,
-0.0724288896,
0.2753514946,
0.0008794446,
-0.0096098054,
0.3409214616,
0.0701248795,
0.6287547946,
0.5038678646,
-0.1901981533,
0.167904824,
-0.0031842482,
-0.1370070428,
-0.3670369089,
-0.2273973674,
0.1343926787,
-0.2523147762,
0.1493882239,
0.4214679301,
-0.0082762623,
-0.4179485142,
-0.0153308073,
0.2546685934,
-0.2011985928,
-0.0177168176,
0.1325405389,
-0.1200013757,
0.2148342729,
0.2546667755,
-0.1645082086,
-0.3432221711,
-0.0456091464,
-0.087129809,
-0.108641617,
-0.1062070206,
-0.2582455575,
-0.4892930686,
-0.0094414242,
-0.3960965872,
0.2227959782,
0.0528321527,
0.3650018573,
-0.0304192565,
0.1201307699,
0.3037039042,
0.0031183604,
0.5263445973,
-0.4160198867,
-0.1148831621,
0.1485776901,
-0.3820872605,
-0.3232316673,
0.0533494987,
-0.1624047309,
0.3027198315,
0.5608932972,
0.5175609589,
-0.0368241332,
-0.2009236813,
-0.0143957669,
0.1242439225,
0.1565524936,
-0.2552374899,
-0.3296490908,
0.1101542115,
-0.1132433265,
0.1082987711,
-0.0189094227,
0.1200615391,
0.1201652288,
-0.1573019624,
0.009452288,
0.1104448587,
0.2438210994,
0.2051903158,
0.1092440784,
0.0710770264,
0.3489989042,
0.2189204395,
-0.1075212136,
0.0731960312,
0.3272774518,
-0.1145712435,
-0.1128835082,
0.2355373353,
0.0178016014,
0.3012569547,
0.2909528911,
0.1026962027,
0.0352196209,
0.148835063,
0.2517143488,
-0.2204652876,
0.3065985441,
0.3736084104,
0.3654961586,
-0.4923385084,
-0.5268407464,
-0.0194871929,
0.4596677423,
-0.1460445225,
0.2637673616,
-0.7810161114,
0.0906471461,
0.0490971655,
0.0831262544,
0.7045710683,
-0.433418721,
0.0311703105,
-0.3011369407,
0.2061883211,
-0.0606679879,
-0.5662207007,
0.1323686689,
-0.1896802485,
-0.3490003645,
0.0372540355,
-0.108756952,
0.2165381461,
0.537293911,
0.1653519273,
0.2765434384,
0.3553302586,
0.108491905,
-0.0140683753,
0.3652673662,
0.5064212084,
-0.3291372657,
0.1828555018,
0.0533330627,
0.1820653826,
-0.241664201,
0.0324815735,
0.0963766798,
-0.0506632291,
0.0337358415,
-0.3181340992,
-0.1936037838,
-0.4162678123,
0.2353664339,
-0.0543479584,
0.2807029486,
0.3980903327,
0.2447256893,
-0.0802795738,
0.0983420089,
-0.0073443251,
0.0743071064,
0.2642247081,
0.2403722256,
0.3874530494,
-0.4567796588,
-0.0083355224,
-0.0959097743,
-0.2261184454,
-0.2581938207,
-0.1545785964,
-0.0594801083,
-0.1083790064,
0.4497852623,
0.1455170959,
0.1635870039,
-0.1944186687,
0.2312682122,
0.0901705846,
-0.1244406328,
-0.0003968934,
0.0900223926,
0.0621658973,
0.6624906063,
-0.2686817944,
-0.4959304929,
-0.0242757387,
0.4147471488,
0.2246546,
-0.077073589,
0.0569660924,
0.206865713,
-0.285607338,
-0.1542340964,
0.157570824,
-0.1063688993,
-0.1054042652,
0.1102898046,
-0.0285244528,
-0.2333063632,
0.2675740421,
-0.1570523679,
-0.1613532752,
-0.091972515,
-0.1774331778,
-0.2806317508,
-0.241336152,
-0.1948482692,
-0.3812471032,
-0.2053016722,
0.2162441164,
0.0149362125,
0.1217984781,
0.4578033388,
-0.1445572823,
0.0478688404,
0.0947164968,
0.1962701529,
-0.0460683368,
-0.2353439331,
0.0793063715,
-0.0385893695,
-0.0526576973,
0.1063537002,
-0.0901939496,
-0.1575679183,
-0.0040823617,
0.1757276207,
-0.0131400153,
-0.2532691658,
0.349930793,
-0.1179879978,
-0.2294913381,
-0.4110828042,
-0.0305958427,
0.0631315261,
-0.0195532907,
-0.0836749971,
0.2614878416,
-0.3048561513,
-0.2629295588,
0.4484552741,
-0.1784747392,
-0.0706913471,
-0.0015809744,
0.2966398895,
0.4717695117,
-0.0923743472,
-0.0766381323,
0.0200876724,
0.2456788123,
0.051516708,
0.1467118412,
-0.1209756359,
0.2085913122,
0.2829656899,
0.3256472051,
0.1422912776,
0.10228578,
-0.249879986,
0.0113923987,
0.016536044,
-0.2410699278,
-0.1997713149,
0.5652071834,
0.0460901856,
0.1719611883,
0.0491667353,
0.3464101553,
0.1937987953,
-0.0170079246,
-0.0793522,
0.0941890255,
-0.2903537452,
0.059407942,
0.1839843541,
0.2628832757,
0.2688405514,
0.43619892,
0.1250479668,
-0.0083823744,
0.4837763906,
0.2577883601,
0.31509161,
0.5179548264,
0.2492690235,
-0.0326479524,
-0.3772099316,
0.1907109916,
0.4949611425,
-0.3534460962,
-0.0469897874,
-0.064874582,
0.2101316452,
-0.2522918582,
-0.3471129239,
-0.2580217123,
0.3463009596,
-0.1636480242,
-0.2520793378,
0.2229750007,
-0.0683909282,
-0.0241792295,
0.1769173294,
-0.2295465022,
0.1455717385,
0.7704070807,
0.1165914088,
0.0410268195,
-0.381588012,
-0.3096579611,
-0.1625859886,
0.1533403993,
-0.1430545002,
0.1957414448,
-0.3325935602,
-0.0119431652,
-0.0246118531,
0.3084971905,
0.3674662411,
0.3030282259,
-0.1277543604,
0.082833536,
-0.0116251856,
0.1468792111,
-0.0056863138,
0.1899856925,
-0.0187838208,
0.175365448,
0.1828663945,
-0.0948646665,
-0.092304334,
-0.4366371334,
0.0524753258,
0.3449984193,
-0.164899081,
0.1421356499,
-0.3117670417,
-0.1528323293,
0.0407488123,
0.2989785969,
-0.2570866942,
-0.0971028358,
0.5395146012,
-0.4918874502,
0.0776440278,
-0.167245239,
0.0359826572,
-0.0960044935,
0.5680377483,
0.3039912879,
-0.0151548991,
-0.3837940395,
-0.1259978712,
-0.6268155575,
-0.0605527572,
-0.3254489005,
0.2521608472,
0.0694541708,
0.2867885828,
0.0498370007,
0.1253739297,
0.3945254087,
-0.0954662189,
-0.0745221674,
0.3330538869,
-0.3459300399,
0.4537990093,
-0.2335766256,
-0.2067915052,
-0.0811020732,
-0.4442894757,
0.3849734366,
0.0730765387,
-0.1252093613,
-0.1655891091,
-0.0261919163,
0.1744762957,
0.1303195506,
0.1954288185,
0.3366407454,
0.3453725874,
0.0390877984,
0.0184385478,
-0.1458938718,
0.1976266354,
-0.0389231704,
0.3265100121,
-0.0632226542,
0.0547963828,
-0.2816341519,
0.1722019166,
0.1057349667,
-0.1668166369,
0.0084095849,
-0.2033715695,
-0.3303464055,
-0.038922444,
-0.0294360071,
0.3369323611,
0.0936918184,
0.3858311772,
0.1202972233,
-0.0968829691,
-0.1704242676,
-0.1283486038,
-0.0215359423,
-0.2728498876,
-0.303637743,
-0.4393439889,
0.2135728747,
-0.2672080398,
0.1529792547,
-0.2265082151,
-0.3191963732,
0.3211679757,
-0.0462014601,
-0.3679233193,
0.3406696618,
-0.3830780685,
-0.0795065314,
-0.1634628922,
0.0433351621,
-0.0836591125,
-0.4640286267,
0.1866619885,
-0.1077283025
]
|
https://github.com/huggingface/datasets/issues/1990 | OSError: Memory mapping file failed: Cannot allocate memory | Do you think this is trying to bring the dataset into memory and if I can avoid it to save on memory so it only brings a batch into memory? @lhoestq thank you | Hi,
I am trying to run a code with a wikipedia dataset, here is the command to reproduce the error. You can find the codes for run_mlm.py in huggingface repo here: https://github.com/huggingface/transformers/blob/v4.3.2/examples/language-modeling/run_mlm.py
```
python run_mlm.py --model_name_or_path bert-base-multilingual-cased --dataset_name wikipedia --dataset_config_name 20200501.en --do_train --do_eval --output_dir /dara/test --max_seq_length 128
```
I am using transformer version: 4.3.2
But I got memory erorr using this dataset, is there a way I could save on memory with dataset library with wikipedia dataset?
Specially I need to train a model with multiple of wikipedia datasets concatenated. thank you very much @lhoestq for your help and suggestions:
```
File "run_mlm.py", line 441, in <module>
main()
File "run_mlm.py", line 233, in main
split=f"train[{data_args.validation_split_percentage}%:]",
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/load.py", line 750, in load_dataset
ds = builder_instance.as_dataset(split=split, ignore_verifications=ignore_verifications, in_memory=keep_in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 740, in as_dataset
map_tuple=True,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/utils/py_utils.py", line 225, in map_nested
return function(data_struct)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 757, in _build_single_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 829, in _as_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 215, in read
return self.read_files(files=files, original_instructions=instructions, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 236, in read_files
pa_table = self._read_files(files, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 171, in _read_files
pa_table: pa.Table = self._get_dataset_from_filename(f_dict, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 302, in _get_dataset_from_filename
pa_table = ArrowReader.read_table(filename, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 322, in read_table
stream = stream_from(filename)
File "pyarrow/io.pxi", line 782, in pyarrow.lib.memory_map
File "pyarrow/io.pxi", line 743, in pyarrow.lib.MemoryMappedFile._open
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 99, in pyarrow.lib.check_status
OSError: Memory mapping file failed: Cannot allocate memory
```
| 33 | OSError: Memory mapping file failed: Cannot allocate memory
Hi,
I am trying to run a code with a wikipedia dataset, here is the command to reproduce the error. You can find the codes for run_mlm.py in huggingface repo here: https://github.com/huggingface/transformers/blob/v4.3.2/examples/language-modeling/run_mlm.py
```
python run_mlm.py --model_name_or_path bert-base-multilingual-cased --dataset_name wikipedia --dataset_config_name 20200501.en --do_train --do_eval --output_dir /dara/test --max_seq_length 128
```
I am using transformer version: 4.3.2
But I got memory erorr using this dataset, is there a way I could save on memory with dataset library with wikipedia dataset?
Specially I need to train a model with multiple of wikipedia datasets concatenated. thank you very much @lhoestq for your help and suggestions:
```
File "run_mlm.py", line 441, in <module>
main()
File "run_mlm.py", line 233, in main
split=f"train[{data_args.validation_split_percentage}%:]",
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/load.py", line 750, in load_dataset
ds = builder_instance.as_dataset(split=split, ignore_verifications=ignore_verifications, in_memory=keep_in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 740, in as_dataset
map_tuple=True,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/utils/py_utils.py", line 225, in map_nested
return function(data_struct)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 757, in _build_single_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 829, in _as_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 215, in read
return self.read_files(files=files, original_instructions=instructions, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 236, in read_files
pa_table = self._read_files(files, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 171, in _read_files
pa_table: pa.Table = self._get_dataset_from_filename(f_dict, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 302, in _get_dataset_from_filename
pa_table = ArrowReader.read_table(filename, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 322, in read_table
stream = stream_from(filename)
File "pyarrow/io.pxi", line 782, in pyarrow.lib.memory_map
File "pyarrow/io.pxi", line 743, in pyarrow.lib.MemoryMappedFile._open
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 99, in pyarrow.lib.check_status
OSError: Memory mapping file failed: Cannot allocate memory
```
Do you think this is trying to bring the dataset into memory and if I can avoid it to save on memory so it only brings a batch into memory? @lhoestq thank you | [
-0.2615424395,
-0.0372640304,
0.0520399138,
0.6045719981,
0.4538376331,
0.2834010124,
0.145643726,
0.272467047,
0.178551048,
0.1056608111,
-0.0581840612,
0.2273861319,
-0.1771183163,
-0.1459163874,
-0.0370905027,
-0.1933318675,
0.0840208605,
0.0134712467,
-0.5429400206,
0.1604686975,
-0.3581553102,
0.1157062277,
-0.1856329739,
-0.117159918,
-0.2980373204,
-0.1035161838,
-0.000604235,
-0.0976307914,
0.0188780669,
-0.3274092674,
0.2548560798,
-0.1293894053,
0.0864008665,
0.5501936078,
-0.0001231607,
-0.0137764234,
0.2835690379,
-0.167208463,
-0.2533996701,
-0.1284160018,
-0.0416566841,
-0.0297982302,
0.0984969735,
-0.2252505571,
-0.0119243311,
-0.0525927581,
0.2317304313,
-0.3445149958,
0.4429750741,
0.2243883014,
0.1404945999,
0.0699656308,
0.4524519742,
-0.0441127494,
0.1739070714,
0.3341217339,
0.0713807866,
0.3320227563,
-0.290871352,
-0.4356869757,
-0.030412823,
0.3930504322,
-0.0399407893,
0.0052878782,
0.5653294921,
-0.1445836425,
0.0253529437,
-0.3368179202,
0.1123083234,
-0.0072491751,
0.5341085792,
-0.4902599454,
-0.0283724777,
-0.0147869457,
-0.053383477,
-0.095239982,
0.2970982194,
0.2689524889,
-0.2722629905,
-0.1447606534,
-0.130416736,
-0.3249987662,
-0.3197000623,
0.4428685009,
-0.0909215808,
0.1910010129,
-0.0247158781,
0.3037816286,
0.5653708577,
-0.2050894946,
-0.234061718,
-0.0758121461,
0.1365433037,
0.3287966251,
-0.2677208483,
-0.1660288125,
-0.3029489219,
-0.2047091722,
0.3511369228,
-0.4987059534,
-0.386192888,
-0.1260009706,
0.1516698003,
0.0212389566,
0.403645277,
0.28173545,
-0.2963657677,
0.3228372931,
0.3122967184,
0.1742713451,
-0.2495379746,
-0.1589902043,
0.0767948925,
-0.0373788811,
-0.111049898,
-0.1914829165,
0.0837734789,
-0.0016539734,
-0.0137460567,
-0.0218036976,
-0.2128317654,
-0.1232090816,
-0.0538496077,
0.5055232048,
-0.1040842906,
-0.084619157,
0.3738277555,
0.1444598138,
-0.0126847355,
-0.0080768447,
-0.056374833,
0.4104229212,
-0.3436279893,
0.2843068242,
0.0502637289,
-0.0011585152,
0.3263410032,
-0.0727056116,
-0.0331280567,
-0.0566816367,
0.1620452106,
-0.3580230772,
0.0087108891,
0.1846879125,
0.1788565069,
0.2493289709,
0.250351131,
-0.1479858309,
-0.1526845396,
0.1969968677,
-0.1634679139,
-0.2433035523,
0.0387368388,
0.0109293237,
0.0980136022,
0.2277473658,
-0.2775138617,
0.1828105599,
0.652862072,
-0.0775154158,
-0.057265494,
-0.0801966339,
-0.229668349,
-0.1687687784,
0.2708782256,
0.5193136334,
-0.0646528676,
-0.1054836139,
-0.1169701889,
0.1677044332,
0.2510126233,
0.4413835704,
-0.1638228744,
0.2519157827,
-0.0972216874,
0.0167984273,
0.4141839445,
-0.3105097711,
-0.3419039547,
-0.0056235818,
-0.0252293423,
-0.1343224347,
0.0232780278,
0.1321308911,
0.0773997977,
0.1156656668,
0.1119260341,
0.3104186356,
0.0561035797,
0.2800839841,
-0.3041983843,
-0.2975763381,
0.2528688908,
0.0747461915,
0.0653965995,
-0.2040828168,
-0.0608490184,
0.810767293,
0.2972948253,
-0.2623124719,
0.1555341631,
0.3147749901,
0.1061367616,
0.0064362576,
0.0871190503,
-0.2199127674,
-0.2514472604,
-0.0734245777,
-0.0566989966,
0.3305933177,
-0.1451886147,
-0.0414811112,
0.1537228972,
-0.1186706349,
-0.1722494811,
-0.3908625245,
0.0622953326,
0.0130735496,
0.1235826537,
0.09008459,
0.0810606107,
0.056008704,
-0.0939699188,
0.2478924394,
-0.5998016596,
0.1715028137,
-0.2896076739,
-0.1076941863,
-0.0214398988,
-0.0624916218,
0.0274148695,
-0.0474656932,
-0.0685558096,
0.151992619,
0.0771345571,
-0.1671152562,
-0.0906845629,
0.0034199415,
0.3101094067,
-0.3221139908,
0.1657060385,
0.2421717942,
0.1955044568,
-0.0940227062,
-0.1976960301,
-0.1558781862,
0.1051340252,
0.3647212386,
0.0791877508,
0.1687752157,
0.0262405798,
0.0895476192,
0.1797856688,
-0.2070019245,
0.2181633115,
0.0123555213,
0.2408843637,
0.0950062498,
-0.0293945745,
-0.3097334206,
0.4583242834,
0.2681970894,
0.2772993445,
0.2424815446,
-0.4937293231,
-0.0740423277,
-0.0656516552,
-0.0718549043,
0.3221568167,
0.050078582,
-0.1195638403,
0.1201549098,
0.1963380426,
0.009703177,
0.3097597659,
0.1743883491,
0.4940780401,
-0.0187084917,
0.1057225913,
-0.0806348994,
-0.0995870829,
-0.1468482763,
0.0500457771,
0.507763803,
-0.1753805876,
-0.0157852508,
-0.1864269525,
-0.4006139934,
-0.2753978968,
0.1643144488,
-0.464242667,
-0.1369271427,
-0.384930104,
0.324758172,
0.0695632696,
0.2584399283,
0.4160760045,
-0.0507141761,
0.3513424397,
-0.1406715214,
0.0687717423,
-0.230412364,
-0.1190754846,
-0.0627370998,
0.4409624636,
-0.2204724401,
0.0868327916,
0.1567280591,
-0.3195577264,
-0.2135091126,
-0.1457151026,
0.146989584,
-0.0808213428,
0.1255743355,
0.0596420467,
0.5084346533,
-0.1233983934,
-0.2323761433,
0.1721096337,
0.0899068639,
-0.058872208,
-0.0373006128,
0.0232935697,
0.1553850174,
0.0540890247,
-0.2931140363,
-0.1797574908,
-0.5081845522,
0.4014801979,
-0.0338908918,
0.1513631344,
0.3279176652,
0.1617627889,
0.1156152114,
-0.1213659048,
0.089448072,
-0.1621508747,
0.0160201583,
0.3207913637,
-0.1488260329,
-0.211434871,
-0.0290637761,
0.0627377853,
0.3063040078,
0.2301857173,
-0.6157576442,
-0.0209110267,
-0.1136819571,
-0.0108944923,
-0.0588510372,
0.286311388,
0.3913798928,
0.0789214522,
0.1078000814,
0.1375888139,
-0.1677573621,
0.0949070081,
-0.0409829654,
0.3248966634,
0.2200790942,
0.5234264135,
0.063726522,
0.8384485841,
0.3419641554,
0.187140435,
0.2288258374,
-0.0220659506,
0.0835646093,
0.0049700928,
-0.3481869698,
-0.0082088821,
-0.0368969217,
0.0619997904,
0.1661427766,
0.0087551922,
-0.3374429047,
-0.1676723063,
-0.3505286574,
0.0459903963,
-0.3771667182,
0.2698537111,
0.1751248091,
0.3674234748,
-0.0838354081,
-0.0824134573,
0.0231612474,
-0.3515779972,
0.1945329756,
0.2135324776,
-0.0175285209,
0.0211244822,
-0.1137083545,
-0.3958894312,
-0.6405463219,
0.1182040945,
-0.1544020027,
0.0629795864,
-0.1038482636,
0.0933977216,
0.0600523278,
0.158307448,
0.7553269863,
0.0059234402,
-0.3072927296,
-0.0461269282,
-0.2609418631,
-0.5063740611,
0.2137844414,
-0.1841569394,
0.3441571891,
0.1433893144,
0.5205534697,
-0.3616020083,
-0.0981341898,
0.3212879598,
0.3747146726,
-0.2170826793,
-0.204692319,
-0.1343318969,
-0.2551261783,
-0.6043949723,
0.0041028555,
0.0545694306,
0.1934489608,
0.4964525402,
0.2044561356,
0.0526690781,
-0.0508969165,
0.1371939182,
-0.0533755906,
0.3105923831,
0.1106538773,
0.1008514613,
0.1345039904,
-0.1580678374,
0.3764191866,
0.2939083576,
0.0716901049,
-0.379755348,
-0.0997852832,
0.0576215051,
0.2523964643,
-0.0627553761,
-0.0139148347,
0.0038341142,
0.0268993154,
0.1780953854,
-0.0997750536,
0.1322199404,
0.187568143,
0.0874078646,
-0.4511453807,
-0.4366311729,
0.3414417505,
0.1997043639,
0.1596940011,
0.3301561475,
-0.1424446404,
-0.5566367507,
0.3253409564,
0.3589582145,
0.9608319998,
-0.3551482558,
0.3937278986,
0.0962183401,
0.2117650509,
0.6567655802,
-0.4751399457,
0.35296157,
-0.3699404895,
-0.081575796,
0.0431751236,
-0.1330996454,
0.1379618049,
-0.0172324833,
-0.4360865951,
0.2891325355,
0.0913326368,
-0.0398276001,
-0.1840587556,
0.3883706033,
0.1124473065,
-0.48119995,
-0.1841866821,
0.0319510885,
-0.1269842386,
0.2439667881,
-0.0456033051,
-0.0274405442,
0.115675956,
-0.1150274202,
-0.3924034834,
-0.0374513194,
-0.4836361706,
0.3076910377,
-0.2517832816,
0.0015951523,
0.4047461748,
0.3928067386,
0.0101811597,
0.369848609,
-0.1340499073,
0.0105265826,
-0.3600814641,
-0.2767651975,
-0.185056746,
0.0676644444,
0.2424039692,
-0.1937426329,
-0.1071276665,
0.0763599202,
-0.0399470702,
-0.1982410252,
-0.2103526294,
-0.0318766981,
-0.1298190653,
-0.2045655698,
-0.2123932242,
0.0132842474,
-0.3584756553,
-0.0186202638,
0.0458631888,
0.0108469883,
-0.3378366232,
0.3286833167,
0.1423628628,
-0.4008429945,
0.0307401512,
0.4164580703,
0.3485439122,
0.1605853885,
0.7200255394,
0.323261708,
-0.2456385195,
-0.1618595421,
-0.0824731737,
-0.0253359526,
-0.3048343062,
0.2100924999,
0.0837552622,
0.1060535014,
-0.3066003621,
-0.0003774762,
0.1285433918,
0.0452356935,
0.0363890566,
-0.3043984175,
-0.5601997972,
0.2082496285,
-0.0104781119,
0.0364991426,
0.2368942797,
0.0221907832,
0.0295316279,
0.2480151206,
-0.156630531,
0.0200542696,
-0.1691418737,
0.2507919371,
0.2438192368,
-0.1116869524,
0.1032437086,
-0.0129490774,
-0.0043390519,
0.1593104899,
-0.0150238331,
-0.1269527674,
0.0454817601,
0.1532625705,
-0.0268511418,
-0.2777855098,
-0.0963580906,
-0.543037951,
-0.0158970244,
-0.303994,
0.011127837,
0.0212790836,
-0.1362703741,
0.110977523,
0.2019028813,
-0.273165822,
-0.0630257353,
0.2792617679,
-0.0221644267,
-0.0507524982,
0.1658708006,
0.2017491758,
0.093798995,
-0.1570738405,
-0.5362299681,
0.0558344983,
-0.0843360648,
-0.0266278628,
0.1019935533,
-0.1142850742,
-0.3993760049,
0.3194215596,
0.0904667899,
0.1510136276,
-0.1423276216,
0.2068411559,
0.2004514337,
0.0089689372,
-0.1937268972,
-0.0418561623,
0.330198884,
-0.2920087278,
-0.0505977869,
0.3277902603,
-0.1311672479,
0.0453455485,
-0.0299583618,
0.131158188,
0.3588021398,
-0.0734307617,
0.3226176202,
0.0061582257,
-0.0834841952,
-0.0167206824,
0.1939019263,
0.4057277441,
0.2587029934,
0.2043865174,
-0.1556383669,
0.0376388319,
-0.1058721393,
-0.2548226416,
0.0379431695,
-0.0836716592,
0.1414890736,
0.4189735055,
-0.1775915623,
0.1592200845,
-0.1939132512,
0.1780177057,
0.1845970601,
-0.2745579481,
0.0787767842,
0.2984714806,
-0.2116875499,
0.0464743972,
0.1499888301,
-0.1157813296,
-0.0657881498,
0.1417854428,
0.0771140009,
-0.6087389588,
0.4591957331,
0.1467867196,
-0.2206506431,
-0.1409263164,
0.2773649096,
0.4522538483,
0.0710783973,
-0.3053993285,
0.0575941615,
0.0269877221,
0.1365890056,
-0.3318276703,
0.3094460368,
0.4621621668,
0.2915848494,
-0.1956234574,
0.2535726428,
-0.1581638753,
-0.0126190586,
0.015425398,
-0.0236887503,
0.1164659038,
0.2165058404,
0.1130550504,
-0.0109330351,
-0.0586275011,
-0.1510954201,
0.1630201936,
0.1425204277,
-0.2279163748,
-0.3811418712,
-0.0991924331,
-0.1710889935,
-0.0140400529,
-0.0115698958,
-0.5901708007,
0.2826707363,
0.5286231637,
-0.1380670071,
0.0812101886,
0.0202329364,
0.0137295788,
-0.0319661349,
0.4846237004,
0.2030229568,
-0.0330805741,
-0.4814165831,
-0.3177812994,
-0.4150763154,
0.0241080243,
-0.3297815025,
0.0040631229,
-0.0754074827,
0.1153875366,
-0.0473553129,
0.0465940088,
0.0486276522,
-0.3642247021,
-0.0435177833,
0.1776142716,
-0.1560724527,
-0.2553374767,
-0.1273900121,
0.1787595302,
0.179388836,
-0.1981145144,
0.2631113529,
0.008783441,
-0.1398376077,
-0.3518666327,
0.1338684708,
0.0501968861,
0.1779215187,
0.1576357484,
0.0610057488,
0.3895050287,
0.0040852698,
-0.2422716767,
0.0258158147,
0.0002693438,
-0.0998136923,
0.1413407922,
0.1294570714,
0.3691282868,
-0.4097651839,
-0.3827790916,
-0.4327932894,
0.2897743881,
-0.0637848526,
-0.1197689697,
-0.0350802019,
-0.0376016907,
0.2526534796,
0.0688294843,
0.0636018366,
0.1586665064,
-0.0656889006,
-0.0003349491,
-0.4509043992,
-0.2615719438,
0.4598853588,
-0.4583000839,
-0.3856024146,
-0.0861000121,
-0.0950355083,
-0.1459356695,
-0.1394123882,
-0.7795309424,
0.0910597444,
0.2944115102,
0.095530577,
-0.4224689901,
0.0314958505,
-0.1058145165,
0.1201660037,
-0.1256256849,
0.3365495503,
-0.0900949836,
-0.4325063527,
0.1651303619,
-0.2077715397
]
|
https://github.com/huggingface/datasets/issues/1990 | OSError: Memory mapping file failed: Cannot allocate memory | It's not trying to bring the dataset into memory.
Actually, it's trying to memory map the dataset file, which is different. It allows to load large dataset files without filling up memory.
What dataset did you use to get this error ?
On what OS are you running ? What's your python and pyarrow version ? | Hi,
I am trying to run a code with a wikipedia dataset, here is the command to reproduce the error. You can find the codes for run_mlm.py in huggingface repo here: https://github.com/huggingface/transformers/blob/v4.3.2/examples/language-modeling/run_mlm.py
```
python run_mlm.py --model_name_or_path bert-base-multilingual-cased --dataset_name wikipedia --dataset_config_name 20200501.en --do_train --do_eval --output_dir /dara/test --max_seq_length 128
```
I am using transformer version: 4.3.2
But I got memory erorr using this dataset, is there a way I could save on memory with dataset library with wikipedia dataset?
Specially I need to train a model with multiple of wikipedia datasets concatenated. thank you very much @lhoestq for your help and suggestions:
```
File "run_mlm.py", line 441, in <module>
main()
File "run_mlm.py", line 233, in main
split=f"train[{data_args.validation_split_percentage}%:]",
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/load.py", line 750, in load_dataset
ds = builder_instance.as_dataset(split=split, ignore_verifications=ignore_verifications, in_memory=keep_in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 740, in as_dataset
map_tuple=True,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/utils/py_utils.py", line 225, in map_nested
return function(data_struct)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 757, in _build_single_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 829, in _as_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 215, in read
return self.read_files(files=files, original_instructions=instructions, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 236, in read_files
pa_table = self._read_files(files, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 171, in _read_files
pa_table: pa.Table = self._get_dataset_from_filename(f_dict, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 302, in _get_dataset_from_filename
pa_table = ArrowReader.read_table(filename, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 322, in read_table
stream = stream_from(filename)
File "pyarrow/io.pxi", line 782, in pyarrow.lib.memory_map
File "pyarrow/io.pxi", line 743, in pyarrow.lib.MemoryMappedFile._open
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 99, in pyarrow.lib.check_status
OSError: Memory mapping file failed: Cannot allocate memory
```
| 56 | OSError: Memory mapping file failed: Cannot allocate memory
Hi,
I am trying to run a code with a wikipedia dataset, here is the command to reproduce the error. You can find the codes for run_mlm.py in huggingface repo here: https://github.com/huggingface/transformers/blob/v4.3.2/examples/language-modeling/run_mlm.py
```
python run_mlm.py --model_name_or_path bert-base-multilingual-cased --dataset_name wikipedia --dataset_config_name 20200501.en --do_train --do_eval --output_dir /dara/test --max_seq_length 128
```
I am using transformer version: 4.3.2
But I got memory erorr using this dataset, is there a way I could save on memory with dataset library with wikipedia dataset?
Specially I need to train a model with multiple of wikipedia datasets concatenated. thank you very much @lhoestq for your help and suggestions:
```
File "run_mlm.py", line 441, in <module>
main()
File "run_mlm.py", line 233, in main
split=f"train[{data_args.validation_split_percentage}%:]",
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/load.py", line 750, in load_dataset
ds = builder_instance.as_dataset(split=split, ignore_verifications=ignore_verifications, in_memory=keep_in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 740, in as_dataset
map_tuple=True,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/utils/py_utils.py", line 225, in map_nested
return function(data_struct)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 757, in _build_single_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 829, in _as_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 215, in read
return self.read_files(files=files, original_instructions=instructions, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 236, in read_files
pa_table = self._read_files(files, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 171, in _read_files
pa_table: pa.Table = self._get_dataset_from_filename(f_dict, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 302, in _get_dataset_from_filename
pa_table = ArrowReader.read_table(filename, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 322, in read_table
stream = stream_from(filename)
File "pyarrow/io.pxi", line 782, in pyarrow.lib.memory_map
File "pyarrow/io.pxi", line 743, in pyarrow.lib.MemoryMappedFile._open
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 99, in pyarrow.lib.check_status
OSError: Memory mapping file failed: Cannot allocate memory
```
It's not trying to bring the dataset into memory.
Actually, it's trying to memory map the dataset file, which is different. It allows to load large dataset files without filling up memory.
What dataset did you use to get this error ?
On what OS are you running ? What's your python and pyarrow version ? | [
-0.2615424395,
-0.0372640304,
0.0520399138,
0.6045719981,
0.4538376331,
0.2834010124,
0.145643726,
0.272467047,
0.178551048,
0.1056608111,
-0.0581840612,
0.2273861319,
-0.1771183163,
-0.1459163874,
-0.0370905027,
-0.1933318675,
0.0840208605,
0.0134712467,
-0.5429400206,
0.1604686975,
-0.3581553102,
0.1157062277,
-0.1856329739,
-0.117159918,
-0.2980373204,
-0.1035161838,
-0.000604235,
-0.0976307914,
0.0188780669,
-0.3274092674,
0.2548560798,
-0.1293894053,
0.0864008665,
0.5501936078,
-0.0001231607,
-0.0137764234,
0.2835690379,
-0.167208463,
-0.2533996701,
-0.1284160018,
-0.0416566841,
-0.0297982302,
0.0984969735,
-0.2252505571,
-0.0119243311,
-0.0525927581,
0.2317304313,
-0.3445149958,
0.4429750741,
0.2243883014,
0.1404945999,
0.0699656308,
0.4524519742,
-0.0441127494,
0.1739070714,
0.3341217339,
0.0713807866,
0.3320227563,
-0.290871352,
-0.4356869757,
-0.030412823,
0.3930504322,
-0.0399407893,
0.0052878782,
0.5653294921,
-0.1445836425,
0.0253529437,
-0.3368179202,
0.1123083234,
-0.0072491751,
0.5341085792,
-0.4902599454,
-0.0283724777,
-0.0147869457,
-0.053383477,
-0.095239982,
0.2970982194,
0.2689524889,
-0.2722629905,
-0.1447606534,
-0.130416736,
-0.3249987662,
-0.3197000623,
0.4428685009,
-0.0909215808,
0.1910010129,
-0.0247158781,
0.3037816286,
0.5653708577,
-0.2050894946,
-0.234061718,
-0.0758121461,
0.1365433037,
0.3287966251,
-0.2677208483,
-0.1660288125,
-0.3029489219,
-0.2047091722,
0.3511369228,
-0.4987059534,
-0.386192888,
-0.1260009706,
0.1516698003,
0.0212389566,
0.403645277,
0.28173545,
-0.2963657677,
0.3228372931,
0.3122967184,
0.1742713451,
-0.2495379746,
-0.1589902043,
0.0767948925,
-0.0373788811,
-0.111049898,
-0.1914829165,
0.0837734789,
-0.0016539734,
-0.0137460567,
-0.0218036976,
-0.2128317654,
-0.1232090816,
-0.0538496077,
0.5055232048,
-0.1040842906,
-0.084619157,
0.3738277555,
0.1444598138,
-0.0126847355,
-0.0080768447,
-0.056374833,
0.4104229212,
-0.3436279893,
0.2843068242,
0.0502637289,
-0.0011585152,
0.3263410032,
-0.0727056116,
-0.0331280567,
-0.0566816367,
0.1620452106,
-0.3580230772,
0.0087108891,
0.1846879125,
0.1788565069,
0.2493289709,
0.250351131,
-0.1479858309,
-0.1526845396,
0.1969968677,
-0.1634679139,
-0.2433035523,
0.0387368388,
0.0109293237,
0.0980136022,
0.2277473658,
-0.2775138617,
0.1828105599,
0.652862072,
-0.0775154158,
-0.057265494,
-0.0801966339,
-0.229668349,
-0.1687687784,
0.2708782256,
0.5193136334,
-0.0646528676,
-0.1054836139,
-0.1169701889,
0.1677044332,
0.2510126233,
0.4413835704,
-0.1638228744,
0.2519157827,
-0.0972216874,
0.0167984273,
0.4141839445,
-0.3105097711,
-0.3419039547,
-0.0056235818,
-0.0252293423,
-0.1343224347,
0.0232780278,
0.1321308911,
0.0773997977,
0.1156656668,
0.1119260341,
0.3104186356,
0.0561035797,
0.2800839841,
-0.3041983843,
-0.2975763381,
0.2528688908,
0.0747461915,
0.0653965995,
-0.2040828168,
-0.0608490184,
0.810767293,
0.2972948253,
-0.2623124719,
0.1555341631,
0.3147749901,
0.1061367616,
0.0064362576,
0.0871190503,
-0.2199127674,
-0.2514472604,
-0.0734245777,
-0.0566989966,
0.3305933177,
-0.1451886147,
-0.0414811112,
0.1537228972,
-0.1186706349,
-0.1722494811,
-0.3908625245,
0.0622953326,
0.0130735496,
0.1235826537,
0.09008459,
0.0810606107,
0.056008704,
-0.0939699188,
0.2478924394,
-0.5998016596,
0.1715028137,
-0.2896076739,
-0.1076941863,
-0.0214398988,
-0.0624916218,
0.0274148695,
-0.0474656932,
-0.0685558096,
0.151992619,
0.0771345571,
-0.1671152562,
-0.0906845629,
0.0034199415,
0.3101094067,
-0.3221139908,
0.1657060385,
0.2421717942,
0.1955044568,
-0.0940227062,
-0.1976960301,
-0.1558781862,
0.1051340252,
0.3647212386,
0.0791877508,
0.1687752157,
0.0262405798,
0.0895476192,
0.1797856688,
-0.2070019245,
0.2181633115,
0.0123555213,
0.2408843637,
0.0950062498,
-0.0293945745,
-0.3097334206,
0.4583242834,
0.2681970894,
0.2772993445,
0.2424815446,
-0.4937293231,
-0.0740423277,
-0.0656516552,
-0.0718549043,
0.3221568167,
0.050078582,
-0.1195638403,
0.1201549098,
0.1963380426,
0.009703177,
0.3097597659,
0.1743883491,
0.4940780401,
-0.0187084917,
0.1057225913,
-0.0806348994,
-0.0995870829,
-0.1468482763,
0.0500457771,
0.507763803,
-0.1753805876,
-0.0157852508,
-0.1864269525,
-0.4006139934,
-0.2753978968,
0.1643144488,
-0.464242667,
-0.1369271427,
-0.384930104,
0.324758172,
0.0695632696,
0.2584399283,
0.4160760045,
-0.0507141761,
0.3513424397,
-0.1406715214,
0.0687717423,
-0.230412364,
-0.1190754846,
-0.0627370998,
0.4409624636,
-0.2204724401,
0.0868327916,
0.1567280591,
-0.3195577264,
-0.2135091126,
-0.1457151026,
0.146989584,
-0.0808213428,
0.1255743355,
0.0596420467,
0.5084346533,
-0.1233983934,
-0.2323761433,
0.1721096337,
0.0899068639,
-0.058872208,
-0.0373006128,
0.0232935697,
0.1553850174,
0.0540890247,
-0.2931140363,
-0.1797574908,
-0.5081845522,
0.4014801979,
-0.0338908918,
0.1513631344,
0.3279176652,
0.1617627889,
0.1156152114,
-0.1213659048,
0.089448072,
-0.1621508747,
0.0160201583,
0.3207913637,
-0.1488260329,
-0.211434871,
-0.0290637761,
0.0627377853,
0.3063040078,
0.2301857173,
-0.6157576442,
-0.0209110267,
-0.1136819571,
-0.0108944923,
-0.0588510372,
0.286311388,
0.3913798928,
0.0789214522,
0.1078000814,
0.1375888139,
-0.1677573621,
0.0949070081,
-0.0409829654,
0.3248966634,
0.2200790942,
0.5234264135,
0.063726522,
0.8384485841,
0.3419641554,
0.187140435,
0.2288258374,
-0.0220659506,
0.0835646093,
0.0049700928,
-0.3481869698,
-0.0082088821,
-0.0368969217,
0.0619997904,
0.1661427766,
0.0087551922,
-0.3374429047,
-0.1676723063,
-0.3505286574,
0.0459903963,
-0.3771667182,
0.2698537111,
0.1751248091,
0.3674234748,
-0.0838354081,
-0.0824134573,
0.0231612474,
-0.3515779972,
0.1945329756,
0.2135324776,
-0.0175285209,
0.0211244822,
-0.1137083545,
-0.3958894312,
-0.6405463219,
0.1182040945,
-0.1544020027,
0.0629795864,
-0.1038482636,
0.0933977216,
0.0600523278,
0.158307448,
0.7553269863,
0.0059234402,
-0.3072927296,
-0.0461269282,
-0.2609418631,
-0.5063740611,
0.2137844414,
-0.1841569394,
0.3441571891,
0.1433893144,
0.5205534697,
-0.3616020083,
-0.0981341898,
0.3212879598,
0.3747146726,
-0.2170826793,
-0.204692319,
-0.1343318969,
-0.2551261783,
-0.6043949723,
0.0041028555,
0.0545694306,
0.1934489608,
0.4964525402,
0.2044561356,
0.0526690781,
-0.0508969165,
0.1371939182,
-0.0533755906,
0.3105923831,
0.1106538773,
0.1008514613,
0.1345039904,
-0.1580678374,
0.3764191866,
0.2939083576,
0.0716901049,
-0.379755348,
-0.0997852832,
0.0576215051,
0.2523964643,
-0.0627553761,
-0.0139148347,
0.0038341142,
0.0268993154,
0.1780953854,
-0.0997750536,
0.1322199404,
0.187568143,
0.0874078646,
-0.4511453807,
-0.4366311729,
0.3414417505,
0.1997043639,
0.1596940011,
0.3301561475,
-0.1424446404,
-0.5566367507,
0.3253409564,
0.3589582145,
0.9608319998,
-0.3551482558,
0.3937278986,
0.0962183401,
0.2117650509,
0.6567655802,
-0.4751399457,
0.35296157,
-0.3699404895,
-0.081575796,
0.0431751236,
-0.1330996454,
0.1379618049,
-0.0172324833,
-0.4360865951,
0.2891325355,
0.0913326368,
-0.0398276001,
-0.1840587556,
0.3883706033,
0.1124473065,
-0.48119995,
-0.1841866821,
0.0319510885,
-0.1269842386,
0.2439667881,
-0.0456033051,
-0.0274405442,
0.115675956,
-0.1150274202,
-0.3924034834,
-0.0374513194,
-0.4836361706,
0.3076910377,
-0.2517832816,
0.0015951523,
0.4047461748,
0.3928067386,
0.0101811597,
0.369848609,
-0.1340499073,
0.0105265826,
-0.3600814641,
-0.2767651975,
-0.185056746,
0.0676644444,
0.2424039692,
-0.1937426329,
-0.1071276665,
0.0763599202,
-0.0399470702,
-0.1982410252,
-0.2103526294,
-0.0318766981,
-0.1298190653,
-0.2045655698,
-0.2123932242,
0.0132842474,
-0.3584756553,
-0.0186202638,
0.0458631888,
0.0108469883,
-0.3378366232,
0.3286833167,
0.1423628628,
-0.4008429945,
0.0307401512,
0.4164580703,
0.3485439122,
0.1605853885,
0.7200255394,
0.323261708,
-0.2456385195,
-0.1618595421,
-0.0824731737,
-0.0253359526,
-0.3048343062,
0.2100924999,
0.0837552622,
0.1060535014,
-0.3066003621,
-0.0003774762,
0.1285433918,
0.0452356935,
0.0363890566,
-0.3043984175,
-0.5601997972,
0.2082496285,
-0.0104781119,
0.0364991426,
0.2368942797,
0.0221907832,
0.0295316279,
0.2480151206,
-0.156630531,
0.0200542696,
-0.1691418737,
0.2507919371,
0.2438192368,
-0.1116869524,
0.1032437086,
-0.0129490774,
-0.0043390519,
0.1593104899,
-0.0150238331,
-0.1269527674,
0.0454817601,
0.1532625705,
-0.0268511418,
-0.2777855098,
-0.0963580906,
-0.543037951,
-0.0158970244,
-0.303994,
0.011127837,
0.0212790836,
-0.1362703741,
0.110977523,
0.2019028813,
-0.273165822,
-0.0630257353,
0.2792617679,
-0.0221644267,
-0.0507524982,
0.1658708006,
0.2017491758,
0.093798995,
-0.1570738405,
-0.5362299681,
0.0558344983,
-0.0843360648,
-0.0266278628,
0.1019935533,
-0.1142850742,
-0.3993760049,
0.3194215596,
0.0904667899,
0.1510136276,
-0.1423276216,
0.2068411559,
0.2004514337,
0.0089689372,
-0.1937268972,
-0.0418561623,
0.330198884,
-0.2920087278,
-0.0505977869,
0.3277902603,
-0.1311672479,
0.0453455485,
-0.0299583618,
0.131158188,
0.3588021398,
-0.0734307617,
0.3226176202,
0.0061582257,
-0.0834841952,
-0.0167206824,
0.1939019263,
0.4057277441,
0.2587029934,
0.2043865174,
-0.1556383669,
0.0376388319,
-0.1058721393,
-0.2548226416,
0.0379431695,
-0.0836716592,
0.1414890736,
0.4189735055,
-0.1775915623,
0.1592200845,
-0.1939132512,
0.1780177057,
0.1845970601,
-0.2745579481,
0.0787767842,
0.2984714806,
-0.2116875499,
0.0464743972,
0.1499888301,
-0.1157813296,
-0.0657881498,
0.1417854428,
0.0771140009,
-0.6087389588,
0.4591957331,
0.1467867196,
-0.2206506431,
-0.1409263164,
0.2773649096,
0.4522538483,
0.0710783973,
-0.3053993285,
0.0575941615,
0.0269877221,
0.1365890056,
-0.3318276703,
0.3094460368,
0.4621621668,
0.2915848494,
-0.1956234574,
0.2535726428,
-0.1581638753,
-0.0126190586,
0.015425398,
-0.0236887503,
0.1164659038,
0.2165058404,
0.1130550504,
-0.0109330351,
-0.0586275011,
-0.1510954201,
0.1630201936,
0.1425204277,
-0.2279163748,
-0.3811418712,
-0.0991924331,
-0.1710889935,
-0.0140400529,
-0.0115698958,
-0.5901708007,
0.2826707363,
0.5286231637,
-0.1380670071,
0.0812101886,
0.0202329364,
0.0137295788,
-0.0319661349,
0.4846237004,
0.2030229568,
-0.0330805741,
-0.4814165831,
-0.3177812994,
-0.4150763154,
0.0241080243,
-0.3297815025,
0.0040631229,
-0.0754074827,
0.1153875366,
-0.0473553129,
0.0465940088,
0.0486276522,
-0.3642247021,
-0.0435177833,
0.1776142716,
-0.1560724527,
-0.2553374767,
-0.1273900121,
0.1787595302,
0.179388836,
-0.1981145144,
0.2631113529,
0.008783441,
-0.1398376077,
-0.3518666327,
0.1338684708,
0.0501968861,
0.1779215187,
0.1576357484,
0.0610057488,
0.3895050287,
0.0040852698,
-0.2422716767,
0.0258158147,
0.0002693438,
-0.0998136923,
0.1413407922,
0.1294570714,
0.3691282868,
-0.4097651839,
-0.3827790916,
-0.4327932894,
0.2897743881,
-0.0637848526,
-0.1197689697,
-0.0350802019,
-0.0376016907,
0.2526534796,
0.0688294843,
0.0636018366,
0.1586665064,
-0.0656889006,
-0.0003349491,
-0.4509043992,
-0.2615719438,
0.4598853588,
-0.4583000839,
-0.3856024146,
-0.0861000121,
-0.0950355083,
-0.1459356695,
-0.1394123882,
-0.7795309424,
0.0910597444,
0.2944115102,
0.095530577,
-0.4224689901,
0.0314958505,
-0.1058145165,
0.1201660037,
-0.1256256849,
0.3365495503,
-0.0900949836,
-0.4325063527,
0.1651303619,
-0.2077715397
]
|
https://github.com/huggingface/datasets/issues/1990 | OSError: Memory mapping file failed: Cannot allocate memory | Dear @lhoestq
thank you so much for coming back to me. Please find info below:
1) Dataset name: I used wikipedia with config 20200501.en
2) I got these pyarrow in my environment:
pyarrow 2.0.0 <pip>
pyarrow 3.0.0 <pip>
3) python version 3.7.10
4) OS version
lsb_release -a
No LSB modules are available.
Distributor ID: Debian
Description: Debian GNU/Linux 10 (buster)
Release: 10
Codename: buster
Is there a way I could solve the memory issue and if I could run this model, I am using GeForce GTX 108,
thanks
| Hi,
I am trying to run a code with a wikipedia dataset, here is the command to reproduce the error. You can find the codes for run_mlm.py in huggingface repo here: https://github.com/huggingface/transformers/blob/v4.3.2/examples/language-modeling/run_mlm.py
```
python run_mlm.py --model_name_or_path bert-base-multilingual-cased --dataset_name wikipedia --dataset_config_name 20200501.en --do_train --do_eval --output_dir /dara/test --max_seq_length 128
```
I am using transformer version: 4.3.2
But I got memory erorr using this dataset, is there a way I could save on memory with dataset library with wikipedia dataset?
Specially I need to train a model with multiple of wikipedia datasets concatenated. thank you very much @lhoestq for your help and suggestions:
```
File "run_mlm.py", line 441, in <module>
main()
File "run_mlm.py", line 233, in main
split=f"train[{data_args.validation_split_percentage}%:]",
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/load.py", line 750, in load_dataset
ds = builder_instance.as_dataset(split=split, ignore_verifications=ignore_verifications, in_memory=keep_in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 740, in as_dataset
map_tuple=True,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/utils/py_utils.py", line 225, in map_nested
return function(data_struct)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 757, in _build_single_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 829, in _as_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 215, in read
return self.read_files(files=files, original_instructions=instructions, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 236, in read_files
pa_table = self._read_files(files, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 171, in _read_files
pa_table: pa.Table = self._get_dataset_from_filename(f_dict, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 302, in _get_dataset_from_filename
pa_table = ArrowReader.read_table(filename, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 322, in read_table
stream = stream_from(filename)
File "pyarrow/io.pxi", line 782, in pyarrow.lib.memory_map
File "pyarrow/io.pxi", line 743, in pyarrow.lib.MemoryMappedFile._open
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 99, in pyarrow.lib.check_status
OSError: Memory mapping file failed: Cannot allocate memory
```
| 88 | OSError: Memory mapping file failed: Cannot allocate memory
Hi,
I am trying to run a code with a wikipedia dataset, here is the command to reproduce the error. You can find the codes for run_mlm.py in huggingface repo here: https://github.com/huggingface/transformers/blob/v4.3.2/examples/language-modeling/run_mlm.py
```
python run_mlm.py --model_name_or_path bert-base-multilingual-cased --dataset_name wikipedia --dataset_config_name 20200501.en --do_train --do_eval --output_dir /dara/test --max_seq_length 128
```
I am using transformer version: 4.3.2
But I got memory erorr using this dataset, is there a way I could save on memory with dataset library with wikipedia dataset?
Specially I need to train a model with multiple of wikipedia datasets concatenated. thank you very much @lhoestq for your help and suggestions:
```
File "run_mlm.py", line 441, in <module>
main()
File "run_mlm.py", line 233, in main
split=f"train[{data_args.validation_split_percentage}%:]",
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/load.py", line 750, in load_dataset
ds = builder_instance.as_dataset(split=split, ignore_verifications=ignore_verifications, in_memory=keep_in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 740, in as_dataset
map_tuple=True,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/utils/py_utils.py", line 225, in map_nested
return function(data_struct)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 757, in _build_single_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 829, in _as_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 215, in read
return self.read_files(files=files, original_instructions=instructions, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 236, in read_files
pa_table = self._read_files(files, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 171, in _read_files
pa_table: pa.Table = self._get_dataset_from_filename(f_dict, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 302, in _get_dataset_from_filename
pa_table = ArrowReader.read_table(filename, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 322, in read_table
stream = stream_from(filename)
File "pyarrow/io.pxi", line 782, in pyarrow.lib.memory_map
File "pyarrow/io.pxi", line 743, in pyarrow.lib.MemoryMappedFile._open
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 99, in pyarrow.lib.check_status
OSError: Memory mapping file failed: Cannot allocate memory
```
Dear @lhoestq
thank you so much for coming back to me. Please find info below:
1) Dataset name: I used wikipedia with config 20200501.en
2) I got these pyarrow in my environment:
pyarrow 2.0.0 <pip>
pyarrow 3.0.0 <pip>
3) python version 3.7.10
4) OS version
lsb_release -a
No LSB modules are available.
Distributor ID: Debian
Description: Debian GNU/Linux 10 (buster)
Release: 10
Codename: buster
Is there a way I could solve the memory issue and if I could run this model, I am using GeForce GTX 108,
thanks
| [
-0.2615424395,
-0.0372640304,
0.0520399138,
0.6045719981,
0.4538376331,
0.2834010124,
0.145643726,
0.272467047,
0.178551048,
0.1056608111,
-0.0581840612,
0.2273861319,
-0.1771183163,
-0.1459163874,
-0.0370905027,
-0.1933318675,
0.0840208605,
0.0134712467,
-0.5429400206,
0.1604686975,
-0.3581553102,
0.1157062277,
-0.1856329739,
-0.117159918,
-0.2980373204,
-0.1035161838,
-0.000604235,
-0.0976307914,
0.0188780669,
-0.3274092674,
0.2548560798,
-0.1293894053,
0.0864008665,
0.5501936078,
-0.0001231607,
-0.0137764234,
0.2835690379,
-0.167208463,
-0.2533996701,
-0.1284160018,
-0.0416566841,
-0.0297982302,
0.0984969735,
-0.2252505571,
-0.0119243311,
-0.0525927581,
0.2317304313,
-0.3445149958,
0.4429750741,
0.2243883014,
0.1404945999,
0.0699656308,
0.4524519742,
-0.0441127494,
0.1739070714,
0.3341217339,
0.0713807866,
0.3320227563,
-0.290871352,
-0.4356869757,
-0.030412823,
0.3930504322,
-0.0399407893,
0.0052878782,
0.5653294921,
-0.1445836425,
0.0253529437,
-0.3368179202,
0.1123083234,
-0.0072491751,
0.5341085792,
-0.4902599454,
-0.0283724777,
-0.0147869457,
-0.053383477,
-0.095239982,
0.2970982194,
0.2689524889,
-0.2722629905,
-0.1447606534,
-0.130416736,
-0.3249987662,
-0.3197000623,
0.4428685009,
-0.0909215808,
0.1910010129,
-0.0247158781,
0.3037816286,
0.5653708577,
-0.2050894946,
-0.234061718,
-0.0758121461,
0.1365433037,
0.3287966251,
-0.2677208483,
-0.1660288125,
-0.3029489219,
-0.2047091722,
0.3511369228,
-0.4987059534,
-0.386192888,
-0.1260009706,
0.1516698003,
0.0212389566,
0.403645277,
0.28173545,
-0.2963657677,
0.3228372931,
0.3122967184,
0.1742713451,
-0.2495379746,
-0.1589902043,
0.0767948925,
-0.0373788811,
-0.111049898,
-0.1914829165,
0.0837734789,
-0.0016539734,
-0.0137460567,
-0.0218036976,
-0.2128317654,
-0.1232090816,
-0.0538496077,
0.5055232048,
-0.1040842906,
-0.084619157,
0.3738277555,
0.1444598138,
-0.0126847355,
-0.0080768447,
-0.056374833,
0.4104229212,
-0.3436279893,
0.2843068242,
0.0502637289,
-0.0011585152,
0.3263410032,
-0.0727056116,
-0.0331280567,
-0.0566816367,
0.1620452106,
-0.3580230772,
0.0087108891,
0.1846879125,
0.1788565069,
0.2493289709,
0.250351131,
-0.1479858309,
-0.1526845396,
0.1969968677,
-0.1634679139,
-0.2433035523,
0.0387368388,
0.0109293237,
0.0980136022,
0.2277473658,
-0.2775138617,
0.1828105599,
0.652862072,
-0.0775154158,
-0.057265494,
-0.0801966339,
-0.229668349,
-0.1687687784,
0.2708782256,
0.5193136334,
-0.0646528676,
-0.1054836139,
-0.1169701889,
0.1677044332,
0.2510126233,
0.4413835704,
-0.1638228744,
0.2519157827,
-0.0972216874,
0.0167984273,
0.4141839445,
-0.3105097711,
-0.3419039547,
-0.0056235818,
-0.0252293423,
-0.1343224347,
0.0232780278,
0.1321308911,
0.0773997977,
0.1156656668,
0.1119260341,
0.3104186356,
0.0561035797,
0.2800839841,
-0.3041983843,
-0.2975763381,
0.2528688908,
0.0747461915,
0.0653965995,
-0.2040828168,
-0.0608490184,
0.810767293,
0.2972948253,
-0.2623124719,
0.1555341631,
0.3147749901,
0.1061367616,
0.0064362576,
0.0871190503,
-0.2199127674,
-0.2514472604,
-0.0734245777,
-0.0566989966,
0.3305933177,
-0.1451886147,
-0.0414811112,
0.1537228972,
-0.1186706349,
-0.1722494811,
-0.3908625245,
0.0622953326,
0.0130735496,
0.1235826537,
0.09008459,
0.0810606107,
0.056008704,
-0.0939699188,
0.2478924394,
-0.5998016596,
0.1715028137,
-0.2896076739,
-0.1076941863,
-0.0214398988,
-0.0624916218,
0.0274148695,
-0.0474656932,
-0.0685558096,
0.151992619,
0.0771345571,
-0.1671152562,
-0.0906845629,
0.0034199415,
0.3101094067,
-0.3221139908,
0.1657060385,
0.2421717942,
0.1955044568,
-0.0940227062,
-0.1976960301,
-0.1558781862,
0.1051340252,
0.3647212386,
0.0791877508,
0.1687752157,
0.0262405798,
0.0895476192,
0.1797856688,
-0.2070019245,
0.2181633115,
0.0123555213,
0.2408843637,
0.0950062498,
-0.0293945745,
-0.3097334206,
0.4583242834,
0.2681970894,
0.2772993445,
0.2424815446,
-0.4937293231,
-0.0740423277,
-0.0656516552,
-0.0718549043,
0.3221568167,
0.050078582,
-0.1195638403,
0.1201549098,
0.1963380426,
0.009703177,
0.3097597659,
0.1743883491,
0.4940780401,
-0.0187084917,
0.1057225913,
-0.0806348994,
-0.0995870829,
-0.1468482763,
0.0500457771,
0.507763803,
-0.1753805876,
-0.0157852508,
-0.1864269525,
-0.4006139934,
-0.2753978968,
0.1643144488,
-0.464242667,
-0.1369271427,
-0.384930104,
0.324758172,
0.0695632696,
0.2584399283,
0.4160760045,
-0.0507141761,
0.3513424397,
-0.1406715214,
0.0687717423,
-0.230412364,
-0.1190754846,
-0.0627370998,
0.4409624636,
-0.2204724401,
0.0868327916,
0.1567280591,
-0.3195577264,
-0.2135091126,
-0.1457151026,
0.146989584,
-0.0808213428,
0.1255743355,
0.0596420467,
0.5084346533,
-0.1233983934,
-0.2323761433,
0.1721096337,
0.0899068639,
-0.058872208,
-0.0373006128,
0.0232935697,
0.1553850174,
0.0540890247,
-0.2931140363,
-0.1797574908,
-0.5081845522,
0.4014801979,
-0.0338908918,
0.1513631344,
0.3279176652,
0.1617627889,
0.1156152114,
-0.1213659048,
0.089448072,
-0.1621508747,
0.0160201583,
0.3207913637,
-0.1488260329,
-0.211434871,
-0.0290637761,
0.0627377853,
0.3063040078,
0.2301857173,
-0.6157576442,
-0.0209110267,
-0.1136819571,
-0.0108944923,
-0.0588510372,
0.286311388,
0.3913798928,
0.0789214522,
0.1078000814,
0.1375888139,
-0.1677573621,
0.0949070081,
-0.0409829654,
0.3248966634,
0.2200790942,
0.5234264135,
0.063726522,
0.8384485841,
0.3419641554,
0.187140435,
0.2288258374,
-0.0220659506,
0.0835646093,
0.0049700928,
-0.3481869698,
-0.0082088821,
-0.0368969217,
0.0619997904,
0.1661427766,
0.0087551922,
-0.3374429047,
-0.1676723063,
-0.3505286574,
0.0459903963,
-0.3771667182,
0.2698537111,
0.1751248091,
0.3674234748,
-0.0838354081,
-0.0824134573,
0.0231612474,
-0.3515779972,
0.1945329756,
0.2135324776,
-0.0175285209,
0.0211244822,
-0.1137083545,
-0.3958894312,
-0.6405463219,
0.1182040945,
-0.1544020027,
0.0629795864,
-0.1038482636,
0.0933977216,
0.0600523278,
0.158307448,
0.7553269863,
0.0059234402,
-0.3072927296,
-0.0461269282,
-0.2609418631,
-0.5063740611,
0.2137844414,
-0.1841569394,
0.3441571891,
0.1433893144,
0.5205534697,
-0.3616020083,
-0.0981341898,
0.3212879598,
0.3747146726,
-0.2170826793,
-0.204692319,
-0.1343318969,
-0.2551261783,
-0.6043949723,
0.0041028555,
0.0545694306,
0.1934489608,
0.4964525402,
0.2044561356,
0.0526690781,
-0.0508969165,
0.1371939182,
-0.0533755906,
0.3105923831,
0.1106538773,
0.1008514613,
0.1345039904,
-0.1580678374,
0.3764191866,
0.2939083576,
0.0716901049,
-0.379755348,
-0.0997852832,
0.0576215051,
0.2523964643,
-0.0627553761,
-0.0139148347,
0.0038341142,
0.0268993154,
0.1780953854,
-0.0997750536,
0.1322199404,
0.187568143,
0.0874078646,
-0.4511453807,
-0.4366311729,
0.3414417505,
0.1997043639,
0.1596940011,
0.3301561475,
-0.1424446404,
-0.5566367507,
0.3253409564,
0.3589582145,
0.9608319998,
-0.3551482558,
0.3937278986,
0.0962183401,
0.2117650509,
0.6567655802,
-0.4751399457,
0.35296157,
-0.3699404895,
-0.081575796,
0.0431751236,
-0.1330996454,
0.1379618049,
-0.0172324833,
-0.4360865951,
0.2891325355,
0.0913326368,
-0.0398276001,
-0.1840587556,
0.3883706033,
0.1124473065,
-0.48119995,
-0.1841866821,
0.0319510885,
-0.1269842386,
0.2439667881,
-0.0456033051,
-0.0274405442,
0.115675956,
-0.1150274202,
-0.3924034834,
-0.0374513194,
-0.4836361706,
0.3076910377,
-0.2517832816,
0.0015951523,
0.4047461748,
0.3928067386,
0.0101811597,
0.369848609,
-0.1340499073,
0.0105265826,
-0.3600814641,
-0.2767651975,
-0.185056746,
0.0676644444,
0.2424039692,
-0.1937426329,
-0.1071276665,
0.0763599202,
-0.0399470702,
-0.1982410252,
-0.2103526294,
-0.0318766981,
-0.1298190653,
-0.2045655698,
-0.2123932242,
0.0132842474,
-0.3584756553,
-0.0186202638,
0.0458631888,
0.0108469883,
-0.3378366232,
0.3286833167,
0.1423628628,
-0.4008429945,
0.0307401512,
0.4164580703,
0.3485439122,
0.1605853885,
0.7200255394,
0.323261708,
-0.2456385195,
-0.1618595421,
-0.0824731737,
-0.0253359526,
-0.3048343062,
0.2100924999,
0.0837552622,
0.1060535014,
-0.3066003621,
-0.0003774762,
0.1285433918,
0.0452356935,
0.0363890566,
-0.3043984175,
-0.5601997972,
0.2082496285,
-0.0104781119,
0.0364991426,
0.2368942797,
0.0221907832,
0.0295316279,
0.2480151206,
-0.156630531,
0.0200542696,
-0.1691418737,
0.2507919371,
0.2438192368,
-0.1116869524,
0.1032437086,
-0.0129490774,
-0.0043390519,
0.1593104899,
-0.0150238331,
-0.1269527674,
0.0454817601,
0.1532625705,
-0.0268511418,
-0.2777855098,
-0.0963580906,
-0.543037951,
-0.0158970244,
-0.303994,
0.011127837,
0.0212790836,
-0.1362703741,
0.110977523,
0.2019028813,
-0.273165822,
-0.0630257353,
0.2792617679,
-0.0221644267,
-0.0507524982,
0.1658708006,
0.2017491758,
0.093798995,
-0.1570738405,
-0.5362299681,
0.0558344983,
-0.0843360648,
-0.0266278628,
0.1019935533,
-0.1142850742,
-0.3993760049,
0.3194215596,
0.0904667899,
0.1510136276,
-0.1423276216,
0.2068411559,
0.2004514337,
0.0089689372,
-0.1937268972,
-0.0418561623,
0.330198884,
-0.2920087278,
-0.0505977869,
0.3277902603,
-0.1311672479,
0.0453455485,
-0.0299583618,
0.131158188,
0.3588021398,
-0.0734307617,
0.3226176202,
0.0061582257,
-0.0834841952,
-0.0167206824,
0.1939019263,
0.4057277441,
0.2587029934,
0.2043865174,
-0.1556383669,
0.0376388319,
-0.1058721393,
-0.2548226416,
0.0379431695,
-0.0836716592,
0.1414890736,
0.4189735055,
-0.1775915623,
0.1592200845,
-0.1939132512,
0.1780177057,
0.1845970601,
-0.2745579481,
0.0787767842,
0.2984714806,
-0.2116875499,
0.0464743972,
0.1499888301,
-0.1157813296,
-0.0657881498,
0.1417854428,
0.0771140009,
-0.6087389588,
0.4591957331,
0.1467867196,
-0.2206506431,
-0.1409263164,
0.2773649096,
0.4522538483,
0.0710783973,
-0.3053993285,
0.0575941615,
0.0269877221,
0.1365890056,
-0.3318276703,
0.3094460368,
0.4621621668,
0.2915848494,
-0.1956234574,
0.2535726428,
-0.1581638753,
-0.0126190586,
0.015425398,
-0.0236887503,
0.1164659038,
0.2165058404,
0.1130550504,
-0.0109330351,
-0.0586275011,
-0.1510954201,
0.1630201936,
0.1425204277,
-0.2279163748,
-0.3811418712,
-0.0991924331,
-0.1710889935,
-0.0140400529,
-0.0115698958,
-0.5901708007,
0.2826707363,
0.5286231637,
-0.1380670071,
0.0812101886,
0.0202329364,
0.0137295788,
-0.0319661349,
0.4846237004,
0.2030229568,
-0.0330805741,
-0.4814165831,
-0.3177812994,
-0.4150763154,
0.0241080243,
-0.3297815025,
0.0040631229,
-0.0754074827,
0.1153875366,
-0.0473553129,
0.0465940088,
0.0486276522,
-0.3642247021,
-0.0435177833,
0.1776142716,
-0.1560724527,
-0.2553374767,
-0.1273900121,
0.1787595302,
0.179388836,
-0.1981145144,
0.2631113529,
0.008783441,
-0.1398376077,
-0.3518666327,
0.1338684708,
0.0501968861,
0.1779215187,
0.1576357484,
0.0610057488,
0.3895050287,
0.0040852698,
-0.2422716767,
0.0258158147,
0.0002693438,
-0.0998136923,
0.1413407922,
0.1294570714,
0.3691282868,
-0.4097651839,
-0.3827790916,
-0.4327932894,
0.2897743881,
-0.0637848526,
-0.1197689697,
-0.0350802019,
-0.0376016907,
0.2526534796,
0.0688294843,
0.0636018366,
0.1586665064,
-0.0656889006,
-0.0003349491,
-0.4509043992,
-0.2615719438,
0.4598853588,
-0.4583000839,
-0.3856024146,
-0.0861000121,
-0.0950355083,
-0.1459356695,
-0.1394123882,
-0.7795309424,
0.0910597444,
0.2944115102,
0.095530577,
-0.4224689901,
0.0314958505,
-0.1058145165,
0.1201660037,
-0.1256256849,
0.3365495503,
-0.0900949836,
-0.4325063527,
0.1651303619,
-0.2077715397
]
|
https://github.com/huggingface/datasets/issues/1990 | OSError: Memory mapping file failed: Cannot allocate memory | I noticed that the error happens when loading the validation dataset.
What value of `data_args.validation_split_percentage` did you use ? | Hi,
I am trying to run a code with a wikipedia dataset, here is the command to reproduce the error. You can find the codes for run_mlm.py in huggingface repo here: https://github.com/huggingface/transformers/blob/v4.3.2/examples/language-modeling/run_mlm.py
```
python run_mlm.py --model_name_or_path bert-base-multilingual-cased --dataset_name wikipedia --dataset_config_name 20200501.en --do_train --do_eval --output_dir /dara/test --max_seq_length 128
```
I am using transformer version: 4.3.2
But I got memory erorr using this dataset, is there a way I could save on memory with dataset library with wikipedia dataset?
Specially I need to train a model with multiple of wikipedia datasets concatenated. thank you very much @lhoestq for your help and suggestions:
```
File "run_mlm.py", line 441, in <module>
main()
File "run_mlm.py", line 233, in main
split=f"train[{data_args.validation_split_percentage}%:]",
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/load.py", line 750, in load_dataset
ds = builder_instance.as_dataset(split=split, ignore_verifications=ignore_verifications, in_memory=keep_in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 740, in as_dataset
map_tuple=True,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/utils/py_utils.py", line 225, in map_nested
return function(data_struct)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 757, in _build_single_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 829, in _as_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 215, in read
return self.read_files(files=files, original_instructions=instructions, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 236, in read_files
pa_table = self._read_files(files, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 171, in _read_files
pa_table: pa.Table = self._get_dataset_from_filename(f_dict, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 302, in _get_dataset_from_filename
pa_table = ArrowReader.read_table(filename, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 322, in read_table
stream = stream_from(filename)
File "pyarrow/io.pxi", line 782, in pyarrow.lib.memory_map
File "pyarrow/io.pxi", line 743, in pyarrow.lib.MemoryMappedFile._open
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 99, in pyarrow.lib.check_status
OSError: Memory mapping file failed: Cannot allocate memory
```
| 19 | OSError: Memory mapping file failed: Cannot allocate memory
Hi,
I am trying to run a code with a wikipedia dataset, here is the command to reproduce the error. You can find the codes for run_mlm.py in huggingface repo here: https://github.com/huggingface/transformers/blob/v4.3.2/examples/language-modeling/run_mlm.py
```
python run_mlm.py --model_name_or_path bert-base-multilingual-cased --dataset_name wikipedia --dataset_config_name 20200501.en --do_train --do_eval --output_dir /dara/test --max_seq_length 128
```
I am using transformer version: 4.3.2
But I got memory erorr using this dataset, is there a way I could save on memory with dataset library with wikipedia dataset?
Specially I need to train a model with multiple of wikipedia datasets concatenated. thank you very much @lhoestq for your help and suggestions:
```
File "run_mlm.py", line 441, in <module>
main()
File "run_mlm.py", line 233, in main
split=f"train[{data_args.validation_split_percentage}%:]",
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/load.py", line 750, in load_dataset
ds = builder_instance.as_dataset(split=split, ignore_verifications=ignore_verifications, in_memory=keep_in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 740, in as_dataset
map_tuple=True,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/utils/py_utils.py", line 225, in map_nested
return function(data_struct)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 757, in _build_single_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 829, in _as_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 215, in read
return self.read_files(files=files, original_instructions=instructions, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 236, in read_files
pa_table = self._read_files(files, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 171, in _read_files
pa_table: pa.Table = self._get_dataset_from_filename(f_dict, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 302, in _get_dataset_from_filename
pa_table = ArrowReader.read_table(filename, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 322, in read_table
stream = stream_from(filename)
File "pyarrow/io.pxi", line 782, in pyarrow.lib.memory_map
File "pyarrow/io.pxi", line 743, in pyarrow.lib.MemoryMappedFile._open
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 99, in pyarrow.lib.check_status
OSError: Memory mapping file failed: Cannot allocate memory
```
I noticed that the error happens when loading the validation dataset.
What value of `data_args.validation_split_percentage` did you use ? | [
-0.2615424395,
-0.0372640304,
0.0520399138,
0.6045719981,
0.4538376331,
0.2834010124,
0.145643726,
0.272467047,
0.178551048,
0.1056608111,
-0.0581840612,
0.2273861319,
-0.1771183163,
-0.1459163874,
-0.0370905027,
-0.1933318675,
0.0840208605,
0.0134712467,
-0.5429400206,
0.1604686975,
-0.3581553102,
0.1157062277,
-0.1856329739,
-0.117159918,
-0.2980373204,
-0.1035161838,
-0.000604235,
-0.0976307914,
0.0188780669,
-0.3274092674,
0.2548560798,
-0.1293894053,
0.0864008665,
0.5501936078,
-0.0001231607,
-0.0137764234,
0.2835690379,
-0.167208463,
-0.2533996701,
-0.1284160018,
-0.0416566841,
-0.0297982302,
0.0984969735,
-0.2252505571,
-0.0119243311,
-0.0525927581,
0.2317304313,
-0.3445149958,
0.4429750741,
0.2243883014,
0.1404945999,
0.0699656308,
0.4524519742,
-0.0441127494,
0.1739070714,
0.3341217339,
0.0713807866,
0.3320227563,
-0.290871352,
-0.4356869757,
-0.030412823,
0.3930504322,
-0.0399407893,
0.0052878782,
0.5653294921,
-0.1445836425,
0.0253529437,
-0.3368179202,
0.1123083234,
-0.0072491751,
0.5341085792,
-0.4902599454,
-0.0283724777,
-0.0147869457,
-0.053383477,
-0.095239982,
0.2970982194,
0.2689524889,
-0.2722629905,
-0.1447606534,
-0.130416736,
-0.3249987662,
-0.3197000623,
0.4428685009,
-0.0909215808,
0.1910010129,
-0.0247158781,
0.3037816286,
0.5653708577,
-0.2050894946,
-0.234061718,
-0.0758121461,
0.1365433037,
0.3287966251,
-0.2677208483,
-0.1660288125,
-0.3029489219,
-0.2047091722,
0.3511369228,
-0.4987059534,
-0.386192888,
-0.1260009706,
0.1516698003,
0.0212389566,
0.403645277,
0.28173545,
-0.2963657677,
0.3228372931,
0.3122967184,
0.1742713451,
-0.2495379746,
-0.1589902043,
0.0767948925,
-0.0373788811,
-0.111049898,
-0.1914829165,
0.0837734789,
-0.0016539734,
-0.0137460567,
-0.0218036976,
-0.2128317654,
-0.1232090816,
-0.0538496077,
0.5055232048,
-0.1040842906,
-0.084619157,
0.3738277555,
0.1444598138,
-0.0126847355,
-0.0080768447,
-0.056374833,
0.4104229212,
-0.3436279893,
0.2843068242,
0.0502637289,
-0.0011585152,
0.3263410032,
-0.0727056116,
-0.0331280567,
-0.0566816367,
0.1620452106,
-0.3580230772,
0.0087108891,
0.1846879125,
0.1788565069,
0.2493289709,
0.250351131,
-0.1479858309,
-0.1526845396,
0.1969968677,
-0.1634679139,
-0.2433035523,
0.0387368388,
0.0109293237,
0.0980136022,
0.2277473658,
-0.2775138617,
0.1828105599,
0.652862072,
-0.0775154158,
-0.057265494,
-0.0801966339,
-0.229668349,
-0.1687687784,
0.2708782256,
0.5193136334,
-0.0646528676,
-0.1054836139,
-0.1169701889,
0.1677044332,
0.2510126233,
0.4413835704,
-0.1638228744,
0.2519157827,
-0.0972216874,
0.0167984273,
0.4141839445,
-0.3105097711,
-0.3419039547,
-0.0056235818,
-0.0252293423,
-0.1343224347,
0.0232780278,
0.1321308911,
0.0773997977,
0.1156656668,
0.1119260341,
0.3104186356,
0.0561035797,
0.2800839841,
-0.3041983843,
-0.2975763381,
0.2528688908,
0.0747461915,
0.0653965995,
-0.2040828168,
-0.0608490184,
0.810767293,
0.2972948253,
-0.2623124719,
0.1555341631,
0.3147749901,
0.1061367616,
0.0064362576,
0.0871190503,
-0.2199127674,
-0.2514472604,
-0.0734245777,
-0.0566989966,
0.3305933177,
-0.1451886147,
-0.0414811112,
0.1537228972,
-0.1186706349,
-0.1722494811,
-0.3908625245,
0.0622953326,
0.0130735496,
0.1235826537,
0.09008459,
0.0810606107,
0.056008704,
-0.0939699188,
0.2478924394,
-0.5998016596,
0.1715028137,
-0.2896076739,
-0.1076941863,
-0.0214398988,
-0.0624916218,
0.0274148695,
-0.0474656932,
-0.0685558096,
0.151992619,
0.0771345571,
-0.1671152562,
-0.0906845629,
0.0034199415,
0.3101094067,
-0.3221139908,
0.1657060385,
0.2421717942,
0.1955044568,
-0.0940227062,
-0.1976960301,
-0.1558781862,
0.1051340252,
0.3647212386,
0.0791877508,
0.1687752157,
0.0262405798,
0.0895476192,
0.1797856688,
-0.2070019245,
0.2181633115,
0.0123555213,
0.2408843637,
0.0950062498,
-0.0293945745,
-0.3097334206,
0.4583242834,
0.2681970894,
0.2772993445,
0.2424815446,
-0.4937293231,
-0.0740423277,
-0.0656516552,
-0.0718549043,
0.3221568167,
0.050078582,
-0.1195638403,
0.1201549098,
0.1963380426,
0.009703177,
0.3097597659,
0.1743883491,
0.4940780401,
-0.0187084917,
0.1057225913,
-0.0806348994,
-0.0995870829,
-0.1468482763,
0.0500457771,
0.507763803,
-0.1753805876,
-0.0157852508,
-0.1864269525,
-0.4006139934,
-0.2753978968,
0.1643144488,
-0.464242667,
-0.1369271427,
-0.384930104,
0.324758172,
0.0695632696,
0.2584399283,
0.4160760045,
-0.0507141761,
0.3513424397,
-0.1406715214,
0.0687717423,
-0.230412364,
-0.1190754846,
-0.0627370998,
0.4409624636,
-0.2204724401,
0.0868327916,
0.1567280591,
-0.3195577264,
-0.2135091126,
-0.1457151026,
0.146989584,
-0.0808213428,
0.1255743355,
0.0596420467,
0.5084346533,
-0.1233983934,
-0.2323761433,
0.1721096337,
0.0899068639,
-0.058872208,
-0.0373006128,
0.0232935697,
0.1553850174,
0.0540890247,
-0.2931140363,
-0.1797574908,
-0.5081845522,
0.4014801979,
-0.0338908918,
0.1513631344,
0.3279176652,
0.1617627889,
0.1156152114,
-0.1213659048,
0.089448072,
-0.1621508747,
0.0160201583,
0.3207913637,
-0.1488260329,
-0.211434871,
-0.0290637761,
0.0627377853,
0.3063040078,
0.2301857173,
-0.6157576442,
-0.0209110267,
-0.1136819571,
-0.0108944923,
-0.0588510372,
0.286311388,
0.3913798928,
0.0789214522,
0.1078000814,
0.1375888139,
-0.1677573621,
0.0949070081,
-0.0409829654,
0.3248966634,
0.2200790942,
0.5234264135,
0.063726522,
0.8384485841,
0.3419641554,
0.187140435,
0.2288258374,
-0.0220659506,
0.0835646093,
0.0049700928,
-0.3481869698,
-0.0082088821,
-0.0368969217,
0.0619997904,
0.1661427766,
0.0087551922,
-0.3374429047,
-0.1676723063,
-0.3505286574,
0.0459903963,
-0.3771667182,
0.2698537111,
0.1751248091,
0.3674234748,
-0.0838354081,
-0.0824134573,
0.0231612474,
-0.3515779972,
0.1945329756,
0.2135324776,
-0.0175285209,
0.0211244822,
-0.1137083545,
-0.3958894312,
-0.6405463219,
0.1182040945,
-0.1544020027,
0.0629795864,
-0.1038482636,
0.0933977216,
0.0600523278,
0.158307448,
0.7553269863,
0.0059234402,
-0.3072927296,
-0.0461269282,
-0.2609418631,
-0.5063740611,
0.2137844414,
-0.1841569394,
0.3441571891,
0.1433893144,
0.5205534697,
-0.3616020083,
-0.0981341898,
0.3212879598,
0.3747146726,
-0.2170826793,
-0.204692319,
-0.1343318969,
-0.2551261783,
-0.6043949723,
0.0041028555,
0.0545694306,
0.1934489608,
0.4964525402,
0.2044561356,
0.0526690781,
-0.0508969165,
0.1371939182,
-0.0533755906,
0.3105923831,
0.1106538773,
0.1008514613,
0.1345039904,
-0.1580678374,
0.3764191866,
0.2939083576,
0.0716901049,
-0.379755348,
-0.0997852832,
0.0576215051,
0.2523964643,
-0.0627553761,
-0.0139148347,
0.0038341142,
0.0268993154,
0.1780953854,
-0.0997750536,
0.1322199404,
0.187568143,
0.0874078646,
-0.4511453807,
-0.4366311729,
0.3414417505,
0.1997043639,
0.1596940011,
0.3301561475,
-0.1424446404,
-0.5566367507,
0.3253409564,
0.3589582145,
0.9608319998,
-0.3551482558,
0.3937278986,
0.0962183401,
0.2117650509,
0.6567655802,
-0.4751399457,
0.35296157,
-0.3699404895,
-0.081575796,
0.0431751236,
-0.1330996454,
0.1379618049,
-0.0172324833,
-0.4360865951,
0.2891325355,
0.0913326368,
-0.0398276001,
-0.1840587556,
0.3883706033,
0.1124473065,
-0.48119995,
-0.1841866821,
0.0319510885,
-0.1269842386,
0.2439667881,
-0.0456033051,
-0.0274405442,
0.115675956,
-0.1150274202,
-0.3924034834,
-0.0374513194,
-0.4836361706,
0.3076910377,
-0.2517832816,
0.0015951523,
0.4047461748,
0.3928067386,
0.0101811597,
0.369848609,
-0.1340499073,
0.0105265826,
-0.3600814641,
-0.2767651975,
-0.185056746,
0.0676644444,
0.2424039692,
-0.1937426329,
-0.1071276665,
0.0763599202,
-0.0399470702,
-0.1982410252,
-0.2103526294,
-0.0318766981,
-0.1298190653,
-0.2045655698,
-0.2123932242,
0.0132842474,
-0.3584756553,
-0.0186202638,
0.0458631888,
0.0108469883,
-0.3378366232,
0.3286833167,
0.1423628628,
-0.4008429945,
0.0307401512,
0.4164580703,
0.3485439122,
0.1605853885,
0.7200255394,
0.323261708,
-0.2456385195,
-0.1618595421,
-0.0824731737,
-0.0253359526,
-0.3048343062,
0.2100924999,
0.0837552622,
0.1060535014,
-0.3066003621,
-0.0003774762,
0.1285433918,
0.0452356935,
0.0363890566,
-0.3043984175,
-0.5601997972,
0.2082496285,
-0.0104781119,
0.0364991426,
0.2368942797,
0.0221907832,
0.0295316279,
0.2480151206,
-0.156630531,
0.0200542696,
-0.1691418737,
0.2507919371,
0.2438192368,
-0.1116869524,
0.1032437086,
-0.0129490774,
-0.0043390519,
0.1593104899,
-0.0150238331,
-0.1269527674,
0.0454817601,
0.1532625705,
-0.0268511418,
-0.2777855098,
-0.0963580906,
-0.543037951,
-0.0158970244,
-0.303994,
0.011127837,
0.0212790836,
-0.1362703741,
0.110977523,
0.2019028813,
-0.273165822,
-0.0630257353,
0.2792617679,
-0.0221644267,
-0.0507524982,
0.1658708006,
0.2017491758,
0.093798995,
-0.1570738405,
-0.5362299681,
0.0558344983,
-0.0843360648,
-0.0266278628,
0.1019935533,
-0.1142850742,
-0.3993760049,
0.3194215596,
0.0904667899,
0.1510136276,
-0.1423276216,
0.2068411559,
0.2004514337,
0.0089689372,
-0.1937268972,
-0.0418561623,
0.330198884,
-0.2920087278,
-0.0505977869,
0.3277902603,
-0.1311672479,
0.0453455485,
-0.0299583618,
0.131158188,
0.3588021398,
-0.0734307617,
0.3226176202,
0.0061582257,
-0.0834841952,
-0.0167206824,
0.1939019263,
0.4057277441,
0.2587029934,
0.2043865174,
-0.1556383669,
0.0376388319,
-0.1058721393,
-0.2548226416,
0.0379431695,
-0.0836716592,
0.1414890736,
0.4189735055,
-0.1775915623,
0.1592200845,
-0.1939132512,
0.1780177057,
0.1845970601,
-0.2745579481,
0.0787767842,
0.2984714806,
-0.2116875499,
0.0464743972,
0.1499888301,
-0.1157813296,
-0.0657881498,
0.1417854428,
0.0771140009,
-0.6087389588,
0.4591957331,
0.1467867196,
-0.2206506431,
-0.1409263164,
0.2773649096,
0.4522538483,
0.0710783973,
-0.3053993285,
0.0575941615,
0.0269877221,
0.1365890056,
-0.3318276703,
0.3094460368,
0.4621621668,
0.2915848494,
-0.1956234574,
0.2535726428,
-0.1581638753,
-0.0126190586,
0.015425398,
-0.0236887503,
0.1164659038,
0.2165058404,
0.1130550504,
-0.0109330351,
-0.0586275011,
-0.1510954201,
0.1630201936,
0.1425204277,
-0.2279163748,
-0.3811418712,
-0.0991924331,
-0.1710889935,
-0.0140400529,
-0.0115698958,
-0.5901708007,
0.2826707363,
0.5286231637,
-0.1380670071,
0.0812101886,
0.0202329364,
0.0137295788,
-0.0319661349,
0.4846237004,
0.2030229568,
-0.0330805741,
-0.4814165831,
-0.3177812994,
-0.4150763154,
0.0241080243,
-0.3297815025,
0.0040631229,
-0.0754074827,
0.1153875366,
-0.0473553129,
0.0465940088,
0.0486276522,
-0.3642247021,
-0.0435177833,
0.1776142716,
-0.1560724527,
-0.2553374767,
-0.1273900121,
0.1787595302,
0.179388836,
-0.1981145144,
0.2631113529,
0.008783441,
-0.1398376077,
-0.3518666327,
0.1338684708,
0.0501968861,
0.1779215187,
0.1576357484,
0.0610057488,
0.3895050287,
0.0040852698,
-0.2422716767,
0.0258158147,
0.0002693438,
-0.0998136923,
0.1413407922,
0.1294570714,
0.3691282868,
-0.4097651839,
-0.3827790916,
-0.4327932894,
0.2897743881,
-0.0637848526,
-0.1197689697,
-0.0350802019,
-0.0376016907,
0.2526534796,
0.0688294843,
0.0636018366,
0.1586665064,
-0.0656889006,
-0.0003349491,
-0.4509043992,
-0.2615719438,
0.4598853588,
-0.4583000839,
-0.3856024146,
-0.0861000121,
-0.0950355083,
-0.1459356695,
-0.1394123882,
-0.7795309424,
0.0910597444,
0.2944115102,
0.095530577,
-0.4224689901,
0.0314958505,
-0.1058145165,
0.1201660037,
-0.1256256849,
0.3365495503,
-0.0900949836,
-0.4325063527,
0.1651303619,
-0.2077715397
]
|
https://github.com/huggingface/datasets/issues/1990 | OSError: Memory mapping file failed: Cannot allocate memory | Dear @lhoestq
thank you very much for the very sharp observation, indeed, this happens there, I use the default value of 5, I basically plan to subsample a part of the large dataset and choose it as validation set. Do you think this is bringing the data into memory during subsampling? Is there a way I could avoid this?
Thank you very much for the great help.
On Mon, Mar 8, 2021 at 11:28 AM Quentin Lhoest ***@***.***>
wrote:
> I noticed that the error happens when loading the validation dataset.
> What value of data_args.validation_split_percentage did you use ?
>
> —
> You are receiving this because you authored the thread.
> Reply to this email directly, view it on GitHub
> <https://github.com/huggingface/datasets/issues/1990#issuecomment-792655644>,
> or unsubscribe
> <https://github.com/notifications/unsubscribe-auth/AS37NMS337ZUJ7HGGVVCCR3TCSREFANCNFSM4YTYAQ2A>
> .
>
| Hi,
I am trying to run a code with a wikipedia dataset, here is the command to reproduce the error. You can find the codes for run_mlm.py in huggingface repo here: https://github.com/huggingface/transformers/blob/v4.3.2/examples/language-modeling/run_mlm.py
```
python run_mlm.py --model_name_or_path bert-base-multilingual-cased --dataset_name wikipedia --dataset_config_name 20200501.en --do_train --do_eval --output_dir /dara/test --max_seq_length 128
```
I am using transformer version: 4.3.2
But I got memory erorr using this dataset, is there a way I could save on memory with dataset library with wikipedia dataset?
Specially I need to train a model with multiple of wikipedia datasets concatenated. thank you very much @lhoestq for your help and suggestions:
```
File "run_mlm.py", line 441, in <module>
main()
File "run_mlm.py", line 233, in main
split=f"train[{data_args.validation_split_percentage}%:]",
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/load.py", line 750, in load_dataset
ds = builder_instance.as_dataset(split=split, ignore_verifications=ignore_verifications, in_memory=keep_in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 740, in as_dataset
map_tuple=True,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/utils/py_utils.py", line 225, in map_nested
return function(data_struct)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 757, in _build_single_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 829, in _as_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 215, in read
return self.read_files(files=files, original_instructions=instructions, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 236, in read_files
pa_table = self._read_files(files, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 171, in _read_files
pa_table: pa.Table = self._get_dataset_from_filename(f_dict, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 302, in _get_dataset_from_filename
pa_table = ArrowReader.read_table(filename, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 322, in read_table
stream = stream_from(filename)
File "pyarrow/io.pxi", line 782, in pyarrow.lib.memory_map
File "pyarrow/io.pxi", line 743, in pyarrow.lib.MemoryMappedFile._open
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 99, in pyarrow.lib.check_status
OSError: Memory mapping file failed: Cannot allocate memory
```
| 133 | OSError: Memory mapping file failed: Cannot allocate memory
Hi,
I am trying to run a code with a wikipedia dataset, here is the command to reproduce the error. You can find the codes for run_mlm.py in huggingface repo here: https://github.com/huggingface/transformers/blob/v4.3.2/examples/language-modeling/run_mlm.py
```
python run_mlm.py --model_name_or_path bert-base-multilingual-cased --dataset_name wikipedia --dataset_config_name 20200501.en --do_train --do_eval --output_dir /dara/test --max_seq_length 128
```
I am using transformer version: 4.3.2
But I got memory erorr using this dataset, is there a way I could save on memory with dataset library with wikipedia dataset?
Specially I need to train a model with multiple of wikipedia datasets concatenated. thank you very much @lhoestq for your help and suggestions:
```
File "run_mlm.py", line 441, in <module>
main()
File "run_mlm.py", line 233, in main
split=f"train[{data_args.validation_split_percentage}%:]",
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/load.py", line 750, in load_dataset
ds = builder_instance.as_dataset(split=split, ignore_verifications=ignore_verifications, in_memory=keep_in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 740, in as_dataset
map_tuple=True,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/utils/py_utils.py", line 225, in map_nested
return function(data_struct)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 757, in _build_single_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 829, in _as_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 215, in read
return self.read_files(files=files, original_instructions=instructions, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 236, in read_files
pa_table = self._read_files(files, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 171, in _read_files
pa_table: pa.Table = self._get_dataset_from_filename(f_dict, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 302, in _get_dataset_from_filename
pa_table = ArrowReader.read_table(filename, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 322, in read_table
stream = stream_from(filename)
File "pyarrow/io.pxi", line 782, in pyarrow.lib.memory_map
File "pyarrow/io.pxi", line 743, in pyarrow.lib.MemoryMappedFile._open
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 99, in pyarrow.lib.check_status
OSError: Memory mapping file failed: Cannot allocate memory
```
Dear @lhoestq
thank you very much for the very sharp observation, indeed, this happens there, I use the default value of 5, I basically plan to subsample a part of the large dataset and choose it as validation set. Do you think this is bringing the data into memory during subsampling? Is there a way I could avoid this?
Thank you very much for the great help.
On Mon, Mar 8, 2021 at 11:28 AM Quentin Lhoest ***@***.***>
wrote:
> I noticed that the error happens when loading the validation dataset.
> What value of data_args.validation_split_percentage did you use ?
>
> —
> You are receiving this because you authored the thread.
> Reply to this email directly, view it on GitHub
> <https://github.com/huggingface/datasets/issues/1990#issuecomment-792655644>,
> or unsubscribe
> <https://github.com/notifications/unsubscribe-auth/AS37NMS337ZUJ7HGGVVCCR3TCSREFANCNFSM4YTYAQ2A>
> .
>
| [
-0.2615424395,
-0.0372640304,
0.0520399138,
0.6045719981,
0.4538376331,
0.2834010124,
0.145643726,
0.272467047,
0.178551048,
0.1056608111,
-0.0581840612,
0.2273861319,
-0.1771183163,
-0.1459163874,
-0.0370905027,
-0.1933318675,
0.0840208605,
0.0134712467,
-0.5429400206,
0.1604686975,
-0.3581553102,
0.1157062277,
-0.1856329739,
-0.117159918,
-0.2980373204,
-0.1035161838,
-0.000604235,
-0.0976307914,
0.0188780669,
-0.3274092674,
0.2548560798,
-0.1293894053,
0.0864008665,
0.5501936078,
-0.0001231607,
-0.0137764234,
0.2835690379,
-0.167208463,
-0.2533996701,
-0.1284160018,
-0.0416566841,
-0.0297982302,
0.0984969735,
-0.2252505571,
-0.0119243311,
-0.0525927581,
0.2317304313,
-0.3445149958,
0.4429750741,
0.2243883014,
0.1404945999,
0.0699656308,
0.4524519742,
-0.0441127494,
0.1739070714,
0.3341217339,
0.0713807866,
0.3320227563,
-0.290871352,
-0.4356869757,
-0.030412823,
0.3930504322,
-0.0399407893,
0.0052878782,
0.5653294921,
-0.1445836425,
0.0253529437,
-0.3368179202,
0.1123083234,
-0.0072491751,
0.5341085792,
-0.4902599454,
-0.0283724777,
-0.0147869457,
-0.053383477,
-0.095239982,
0.2970982194,
0.2689524889,
-0.2722629905,
-0.1447606534,
-0.130416736,
-0.3249987662,
-0.3197000623,
0.4428685009,
-0.0909215808,
0.1910010129,
-0.0247158781,
0.3037816286,
0.5653708577,
-0.2050894946,
-0.234061718,
-0.0758121461,
0.1365433037,
0.3287966251,
-0.2677208483,
-0.1660288125,
-0.3029489219,
-0.2047091722,
0.3511369228,
-0.4987059534,
-0.386192888,
-0.1260009706,
0.1516698003,
0.0212389566,
0.403645277,
0.28173545,
-0.2963657677,
0.3228372931,
0.3122967184,
0.1742713451,
-0.2495379746,
-0.1589902043,
0.0767948925,
-0.0373788811,
-0.111049898,
-0.1914829165,
0.0837734789,
-0.0016539734,
-0.0137460567,
-0.0218036976,
-0.2128317654,
-0.1232090816,
-0.0538496077,
0.5055232048,
-0.1040842906,
-0.084619157,
0.3738277555,
0.1444598138,
-0.0126847355,
-0.0080768447,
-0.056374833,
0.4104229212,
-0.3436279893,
0.2843068242,
0.0502637289,
-0.0011585152,
0.3263410032,
-0.0727056116,
-0.0331280567,
-0.0566816367,
0.1620452106,
-0.3580230772,
0.0087108891,
0.1846879125,
0.1788565069,
0.2493289709,
0.250351131,
-0.1479858309,
-0.1526845396,
0.1969968677,
-0.1634679139,
-0.2433035523,
0.0387368388,
0.0109293237,
0.0980136022,
0.2277473658,
-0.2775138617,
0.1828105599,
0.652862072,
-0.0775154158,
-0.057265494,
-0.0801966339,
-0.229668349,
-0.1687687784,
0.2708782256,
0.5193136334,
-0.0646528676,
-0.1054836139,
-0.1169701889,
0.1677044332,
0.2510126233,
0.4413835704,
-0.1638228744,
0.2519157827,
-0.0972216874,
0.0167984273,
0.4141839445,
-0.3105097711,
-0.3419039547,
-0.0056235818,
-0.0252293423,
-0.1343224347,
0.0232780278,
0.1321308911,
0.0773997977,
0.1156656668,
0.1119260341,
0.3104186356,
0.0561035797,
0.2800839841,
-0.3041983843,
-0.2975763381,
0.2528688908,
0.0747461915,
0.0653965995,
-0.2040828168,
-0.0608490184,
0.810767293,
0.2972948253,
-0.2623124719,
0.1555341631,
0.3147749901,
0.1061367616,
0.0064362576,
0.0871190503,
-0.2199127674,
-0.2514472604,
-0.0734245777,
-0.0566989966,
0.3305933177,
-0.1451886147,
-0.0414811112,
0.1537228972,
-0.1186706349,
-0.1722494811,
-0.3908625245,
0.0622953326,
0.0130735496,
0.1235826537,
0.09008459,
0.0810606107,
0.056008704,
-0.0939699188,
0.2478924394,
-0.5998016596,
0.1715028137,
-0.2896076739,
-0.1076941863,
-0.0214398988,
-0.0624916218,
0.0274148695,
-0.0474656932,
-0.0685558096,
0.151992619,
0.0771345571,
-0.1671152562,
-0.0906845629,
0.0034199415,
0.3101094067,
-0.3221139908,
0.1657060385,
0.2421717942,
0.1955044568,
-0.0940227062,
-0.1976960301,
-0.1558781862,
0.1051340252,
0.3647212386,
0.0791877508,
0.1687752157,
0.0262405798,
0.0895476192,
0.1797856688,
-0.2070019245,
0.2181633115,
0.0123555213,
0.2408843637,
0.0950062498,
-0.0293945745,
-0.3097334206,
0.4583242834,
0.2681970894,
0.2772993445,
0.2424815446,
-0.4937293231,
-0.0740423277,
-0.0656516552,
-0.0718549043,
0.3221568167,
0.050078582,
-0.1195638403,
0.1201549098,
0.1963380426,
0.009703177,
0.3097597659,
0.1743883491,
0.4940780401,
-0.0187084917,
0.1057225913,
-0.0806348994,
-0.0995870829,
-0.1468482763,
0.0500457771,
0.507763803,
-0.1753805876,
-0.0157852508,
-0.1864269525,
-0.4006139934,
-0.2753978968,
0.1643144488,
-0.464242667,
-0.1369271427,
-0.384930104,
0.324758172,
0.0695632696,
0.2584399283,
0.4160760045,
-0.0507141761,
0.3513424397,
-0.1406715214,
0.0687717423,
-0.230412364,
-0.1190754846,
-0.0627370998,
0.4409624636,
-0.2204724401,
0.0868327916,
0.1567280591,
-0.3195577264,
-0.2135091126,
-0.1457151026,
0.146989584,
-0.0808213428,
0.1255743355,
0.0596420467,
0.5084346533,
-0.1233983934,
-0.2323761433,
0.1721096337,
0.0899068639,
-0.058872208,
-0.0373006128,
0.0232935697,
0.1553850174,
0.0540890247,
-0.2931140363,
-0.1797574908,
-0.5081845522,
0.4014801979,
-0.0338908918,
0.1513631344,
0.3279176652,
0.1617627889,
0.1156152114,
-0.1213659048,
0.089448072,
-0.1621508747,
0.0160201583,
0.3207913637,
-0.1488260329,
-0.211434871,
-0.0290637761,
0.0627377853,
0.3063040078,
0.2301857173,
-0.6157576442,
-0.0209110267,
-0.1136819571,
-0.0108944923,
-0.0588510372,
0.286311388,
0.3913798928,
0.0789214522,
0.1078000814,
0.1375888139,
-0.1677573621,
0.0949070081,
-0.0409829654,
0.3248966634,
0.2200790942,
0.5234264135,
0.063726522,
0.8384485841,
0.3419641554,
0.187140435,
0.2288258374,
-0.0220659506,
0.0835646093,
0.0049700928,
-0.3481869698,
-0.0082088821,
-0.0368969217,
0.0619997904,
0.1661427766,
0.0087551922,
-0.3374429047,
-0.1676723063,
-0.3505286574,
0.0459903963,
-0.3771667182,
0.2698537111,
0.1751248091,
0.3674234748,
-0.0838354081,
-0.0824134573,
0.0231612474,
-0.3515779972,
0.1945329756,
0.2135324776,
-0.0175285209,
0.0211244822,
-0.1137083545,
-0.3958894312,
-0.6405463219,
0.1182040945,
-0.1544020027,
0.0629795864,
-0.1038482636,
0.0933977216,
0.0600523278,
0.158307448,
0.7553269863,
0.0059234402,
-0.3072927296,
-0.0461269282,
-0.2609418631,
-0.5063740611,
0.2137844414,
-0.1841569394,
0.3441571891,
0.1433893144,
0.5205534697,
-0.3616020083,
-0.0981341898,
0.3212879598,
0.3747146726,
-0.2170826793,
-0.204692319,
-0.1343318969,
-0.2551261783,
-0.6043949723,
0.0041028555,
0.0545694306,
0.1934489608,
0.4964525402,
0.2044561356,
0.0526690781,
-0.0508969165,
0.1371939182,
-0.0533755906,
0.3105923831,
0.1106538773,
0.1008514613,
0.1345039904,
-0.1580678374,
0.3764191866,
0.2939083576,
0.0716901049,
-0.379755348,
-0.0997852832,
0.0576215051,
0.2523964643,
-0.0627553761,
-0.0139148347,
0.0038341142,
0.0268993154,
0.1780953854,
-0.0997750536,
0.1322199404,
0.187568143,
0.0874078646,
-0.4511453807,
-0.4366311729,
0.3414417505,
0.1997043639,
0.1596940011,
0.3301561475,
-0.1424446404,
-0.5566367507,
0.3253409564,
0.3589582145,
0.9608319998,
-0.3551482558,
0.3937278986,
0.0962183401,
0.2117650509,
0.6567655802,
-0.4751399457,
0.35296157,
-0.3699404895,
-0.081575796,
0.0431751236,
-0.1330996454,
0.1379618049,
-0.0172324833,
-0.4360865951,
0.2891325355,
0.0913326368,
-0.0398276001,
-0.1840587556,
0.3883706033,
0.1124473065,
-0.48119995,
-0.1841866821,
0.0319510885,
-0.1269842386,
0.2439667881,
-0.0456033051,
-0.0274405442,
0.115675956,
-0.1150274202,
-0.3924034834,
-0.0374513194,
-0.4836361706,
0.3076910377,
-0.2517832816,
0.0015951523,
0.4047461748,
0.3928067386,
0.0101811597,
0.369848609,
-0.1340499073,
0.0105265826,
-0.3600814641,
-0.2767651975,
-0.185056746,
0.0676644444,
0.2424039692,
-0.1937426329,
-0.1071276665,
0.0763599202,
-0.0399470702,
-0.1982410252,
-0.2103526294,
-0.0318766981,
-0.1298190653,
-0.2045655698,
-0.2123932242,
0.0132842474,
-0.3584756553,
-0.0186202638,
0.0458631888,
0.0108469883,
-0.3378366232,
0.3286833167,
0.1423628628,
-0.4008429945,
0.0307401512,
0.4164580703,
0.3485439122,
0.1605853885,
0.7200255394,
0.323261708,
-0.2456385195,
-0.1618595421,
-0.0824731737,
-0.0253359526,
-0.3048343062,
0.2100924999,
0.0837552622,
0.1060535014,
-0.3066003621,
-0.0003774762,
0.1285433918,
0.0452356935,
0.0363890566,
-0.3043984175,
-0.5601997972,
0.2082496285,
-0.0104781119,
0.0364991426,
0.2368942797,
0.0221907832,
0.0295316279,
0.2480151206,
-0.156630531,
0.0200542696,
-0.1691418737,
0.2507919371,
0.2438192368,
-0.1116869524,
0.1032437086,
-0.0129490774,
-0.0043390519,
0.1593104899,
-0.0150238331,
-0.1269527674,
0.0454817601,
0.1532625705,
-0.0268511418,
-0.2777855098,
-0.0963580906,
-0.543037951,
-0.0158970244,
-0.303994,
0.011127837,
0.0212790836,
-0.1362703741,
0.110977523,
0.2019028813,
-0.273165822,
-0.0630257353,
0.2792617679,
-0.0221644267,
-0.0507524982,
0.1658708006,
0.2017491758,
0.093798995,
-0.1570738405,
-0.5362299681,
0.0558344983,
-0.0843360648,
-0.0266278628,
0.1019935533,
-0.1142850742,
-0.3993760049,
0.3194215596,
0.0904667899,
0.1510136276,
-0.1423276216,
0.2068411559,
0.2004514337,
0.0089689372,
-0.1937268972,
-0.0418561623,
0.330198884,
-0.2920087278,
-0.0505977869,
0.3277902603,
-0.1311672479,
0.0453455485,
-0.0299583618,
0.131158188,
0.3588021398,
-0.0734307617,
0.3226176202,
0.0061582257,
-0.0834841952,
-0.0167206824,
0.1939019263,
0.4057277441,
0.2587029934,
0.2043865174,
-0.1556383669,
0.0376388319,
-0.1058721393,
-0.2548226416,
0.0379431695,
-0.0836716592,
0.1414890736,
0.4189735055,
-0.1775915623,
0.1592200845,
-0.1939132512,
0.1780177057,
0.1845970601,
-0.2745579481,
0.0787767842,
0.2984714806,
-0.2116875499,
0.0464743972,
0.1499888301,
-0.1157813296,
-0.0657881498,
0.1417854428,
0.0771140009,
-0.6087389588,
0.4591957331,
0.1467867196,
-0.2206506431,
-0.1409263164,
0.2773649096,
0.4522538483,
0.0710783973,
-0.3053993285,
0.0575941615,
0.0269877221,
0.1365890056,
-0.3318276703,
0.3094460368,
0.4621621668,
0.2915848494,
-0.1956234574,
0.2535726428,
-0.1581638753,
-0.0126190586,
0.015425398,
-0.0236887503,
0.1164659038,
0.2165058404,
0.1130550504,
-0.0109330351,
-0.0586275011,
-0.1510954201,
0.1630201936,
0.1425204277,
-0.2279163748,
-0.3811418712,
-0.0991924331,
-0.1710889935,
-0.0140400529,
-0.0115698958,
-0.5901708007,
0.2826707363,
0.5286231637,
-0.1380670071,
0.0812101886,
0.0202329364,
0.0137295788,
-0.0319661349,
0.4846237004,
0.2030229568,
-0.0330805741,
-0.4814165831,
-0.3177812994,
-0.4150763154,
0.0241080243,
-0.3297815025,
0.0040631229,
-0.0754074827,
0.1153875366,
-0.0473553129,
0.0465940088,
0.0486276522,
-0.3642247021,
-0.0435177833,
0.1776142716,
-0.1560724527,
-0.2553374767,
-0.1273900121,
0.1787595302,
0.179388836,
-0.1981145144,
0.2631113529,
0.008783441,
-0.1398376077,
-0.3518666327,
0.1338684708,
0.0501968861,
0.1779215187,
0.1576357484,
0.0610057488,
0.3895050287,
0.0040852698,
-0.2422716767,
0.0258158147,
0.0002693438,
-0.0998136923,
0.1413407922,
0.1294570714,
0.3691282868,
-0.4097651839,
-0.3827790916,
-0.4327932894,
0.2897743881,
-0.0637848526,
-0.1197689697,
-0.0350802019,
-0.0376016907,
0.2526534796,
0.0688294843,
0.0636018366,
0.1586665064,
-0.0656889006,
-0.0003349491,
-0.4509043992,
-0.2615719438,
0.4598853588,
-0.4583000839,
-0.3856024146,
-0.0861000121,
-0.0950355083,
-0.1459356695,
-0.1394123882,
-0.7795309424,
0.0910597444,
0.2944115102,
0.095530577,
-0.4224689901,
0.0314958505,
-0.1058145165,
0.1201660037,
-0.1256256849,
0.3365495503,
-0.0900949836,
-0.4325063527,
0.1651303619,
-0.2077715397
]
|
https://github.com/huggingface/datasets/issues/1990 | OSError: Memory mapping file failed: Cannot allocate memory | Methods like `dataset.shard`, `dataset.train_test_split`, `dataset.select` etc. don't bring the dataset in memory.
The only time when samples are brought to memory is when you access elements via `dataset[0]`, `dataset[:10]`, `dataset["my_column_names"]`.
But it's possible that trying to use those methods to build your validation set doesn't fix the issue since, if I understand correctly, the error happens when when the dataset arrow file is opened (just before the 5% percentage is applied).
Did you try to reproduce this issue in a google colab ? This would be super helpful to investigate why this happened.
Also maybe you can try clearing your cache at `~/.cache/huggingface/datasets` and try again. If the arrow file was corrupted somehow, removing it and rebuilding may fix the issue. | Hi,
I am trying to run a code with a wikipedia dataset, here is the command to reproduce the error. You can find the codes for run_mlm.py in huggingface repo here: https://github.com/huggingface/transformers/blob/v4.3.2/examples/language-modeling/run_mlm.py
```
python run_mlm.py --model_name_or_path bert-base-multilingual-cased --dataset_name wikipedia --dataset_config_name 20200501.en --do_train --do_eval --output_dir /dara/test --max_seq_length 128
```
I am using transformer version: 4.3.2
But I got memory erorr using this dataset, is there a way I could save on memory with dataset library with wikipedia dataset?
Specially I need to train a model with multiple of wikipedia datasets concatenated. thank you very much @lhoestq for your help and suggestions:
```
File "run_mlm.py", line 441, in <module>
main()
File "run_mlm.py", line 233, in main
split=f"train[{data_args.validation_split_percentage}%:]",
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/load.py", line 750, in load_dataset
ds = builder_instance.as_dataset(split=split, ignore_verifications=ignore_verifications, in_memory=keep_in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 740, in as_dataset
map_tuple=True,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/utils/py_utils.py", line 225, in map_nested
return function(data_struct)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 757, in _build_single_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 829, in _as_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 215, in read
return self.read_files(files=files, original_instructions=instructions, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 236, in read_files
pa_table = self._read_files(files, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 171, in _read_files
pa_table: pa.Table = self._get_dataset_from_filename(f_dict, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 302, in _get_dataset_from_filename
pa_table = ArrowReader.read_table(filename, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 322, in read_table
stream = stream_from(filename)
File "pyarrow/io.pxi", line 782, in pyarrow.lib.memory_map
File "pyarrow/io.pxi", line 743, in pyarrow.lib.MemoryMappedFile._open
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 99, in pyarrow.lib.check_status
OSError: Memory mapping file failed: Cannot allocate memory
```
| 121 | OSError: Memory mapping file failed: Cannot allocate memory
Hi,
I am trying to run a code with a wikipedia dataset, here is the command to reproduce the error. You can find the codes for run_mlm.py in huggingface repo here: https://github.com/huggingface/transformers/blob/v4.3.2/examples/language-modeling/run_mlm.py
```
python run_mlm.py --model_name_or_path bert-base-multilingual-cased --dataset_name wikipedia --dataset_config_name 20200501.en --do_train --do_eval --output_dir /dara/test --max_seq_length 128
```
I am using transformer version: 4.3.2
But I got memory erorr using this dataset, is there a way I could save on memory with dataset library with wikipedia dataset?
Specially I need to train a model with multiple of wikipedia datasets concatenated. thank you very much @lhoestq for your help and suggestions:
```
File "run_mlm.py", line 441, in <module>
main()
File "run_mlm.py", line 233, in main
split=f"train[{data_args.validation_split_percentage}%:]",
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/load.py", line 750, in load_dataset
ds = builder_instance.as_dataset(split=split, ignore_verifications=ignore_verifications, in_memory=keep_in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 740, in as_dataset
map_tuple=True,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/utils/py_utils.py", line 225, in map_nested
return function(data_struct)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 757, in _build_single_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 829, in _as_dataset
in_memory=in_memory,
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 215, in read
return self.read_files(files=files, original_instructions=instructions, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 236, in read_files
pa_table = self._read_files(files, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 171, in _read_files
pa_table: pa.Table = self._get_dataset_from_filename(f_dict, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 302, in _get_dataset_from_filename
pa_table = ArrowReader.read_table(filename, in_memory=in_memory)
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 322, in read_table
stream = stream_from(filename)
File "pyarrow/io.pxi", line 782, in pyarrow.lib.memory_map
File "pyarrow/io.pxi", line 743, in pyarrow.lib.MemoryMappedFile._open
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 99, in pyarrow.lib.check_status
OSError: Memory mapping file failed: Cannot allocate memory
```
Methods like `dataset.shard`, `dataset.train_test_split`, `dataset.select` etc. don't bring the dataset in memory.
The only time when samples are brought to memory is when you access elements via `dataset[0]`, `dataset[:10]`, `dataset["my_column_names"]`.
But it's possible that trying to use those methods to build your validation set doesn't fix the issue since, if I understand correctly, the error happens when when the dataset arrow file is opened (just before the 5% percentage is applied).
Did you try to reproduce this issue in a google colab ? This would be super helpful to investigate why this happened.
Also maybe you can try clearing your cache at `~/.cache/huggingface/datasets` and try again. If the arrow file was corrupted somehow, removing it and rebuilding may fix the issue. | [
-0.2615424395,
-0.0372640304,
0.0520399138,
0.6045719981,
0.4538376331,
0.2834010124,
0.145643726,
0.272467047,
0.178551048,
0.1056608111,
-0.0581840612,
0.2273861319,
-0.1771183163,
-0.1459163874,
-0.0370905027,
-0.1933318675,
0.0840208605,
0.0134712467,
-0.5429400206,
0.1604686975,
-0.3581553102,
0.1157062277,
-0.1856329739,
-0.117159918,
-0.2980373204,
-0.1035161838,
-0.000604235,
-0.0976307914,
0.0188780669,
-0.3274092674,
0.2548560798,
-0.1293894053,
0.0864008665,
0.5501936078,
-0.0001231607,
-0.0137764234,
0.2835690379,
-0.167208463,
-0.2533996701,
-0.1284160018,
-0.0416566841,
-0.0297982302,
0.0984969735,
-0.2252505571,
-0.0119243311,
-0.0525927581,
0.2317304313,
-0.3445149958,
0.4429750741,
0.2243883014,
0.1404945999,
0.0699656308,
0.4524519742,
-0.0441127494,
0.1739070714,
0.3341217339,
0.0713807866,
0.3320227563,
-0.290871352,
-0.4356869757,
-0.030412823,
0.3930504322,
-0.0399407893,
0.0052878782,
0.5653294921,
-0.1445836425,
0.0253529437,
-0.3368179202,
0.1123083234,
-0.0072491751,
0.5341085792,
-0.4902599454,
-0.0283724777,
-0.0147869457,
-0.053383477,
-0.095239982,
0.2970982194,
0.2689524889,
-0.2722629905,
-0.1447606534,
-0.130416736,
-0.3249987662,
-0.3197000623,
0.4428685009,
-0.0909215808,
0.1910010129,
-0.0247158781,
0.3037816286,
0.5653708577,
-0.2050894946,
-0.234061718,
-0.0758121461,
0.1365433037,
0.3287966251,
-0.2677208483,
-0.1660288125,
-0.3029489219,
-0.2047091722,
0.3511369228,
-0.4987059534,
-0.386192888,
-0.1260009706,
0.1516698003,
0.0212389566,
0.403645277,
0.28173545,
-0.2963657677,
0.3228372931,
0.3122967184,
0.1742713451,
-0.2495379746,
-0.1589902043,
0.0767948925,
-0.0373788811,
-0.111049898,
-0.1914829165,
0.0837734789,
-0.0016539734,
-0.0137460567,
-0.0218036976,
-0.2128317654,
-0.1232090816,
-0.0538496077,
0.5055232048,
-0.1040842906,
-0.084619157,
0.3738277555,
0.1444598138,
-0.0126847355,
-0.0080768447,
-0.056374833,
0.4104229212,
-0.3436279893,
0.2843068242,
0.0502637289,
-0.0011585152,
0.3263410032,
-0.0727056116,
-0.0331280567,
-0.0566816367,
0.1620452106,
-0.3580230772,
0.0087108891,
0.1846879125,
0.1788565069,
0.2493289709,
0.250351131,
-0.1479858309,
-0.1526845396,
0.1969968677,
-0.1634679139,
-0.2433035523,
0.0387368388,
0.0109293237,
0.0980136022,
0.2277473658,
-0.2775138617,
0.1828105599,
0.652862072,
-0.0775154158,
-0.057265494,
-0.0801966339,
-0.229668349,
-0.1687687784,
0.2708782256,
0.5193136334,
-0.0646528676,
-0.1054836139,
-0.1169701889,
0.1677044332,
0.2510126233,
0.4413835704,
-0.1638228744,
0.2519157827,
-0.0972216874,
0.0167984273,
0.4141839445,
-0.3105097711,
-0.3419039547,
-0.0056235818,
-0.0252293423,
-0.1343224347,
0.0232780278,
0.1321308911,
0.0773997977,
0.1156656668,
0.1119260341,
0.3104186356,
0.0561035797,
0.2800839841,
-0.3041983843,
-0.2975763381,
0.2528688908,
0.0747461915,
0.0653965995,
-0.2040828168,
-0.0608490184,
0.810767293,
0.2972948253,
-0.2623124719,
0.1555341631,
0.3147749901,
0.1061367616,
0.0064362576,
0.0871190503,
-0.2199127674,
-0.2514472604,
-0.0734245777,
-0.0566989966,
0.3305933177,
-0.1451886147,
-0.0414811112,
0.1537228972,
-0.1186706349,
-0.1722494811,
-0.3908625245,
0.0622953326,
0.0130735496,
0.1235826537,
0.09008459,
0.0810606107,
0.056008704,
-0.0939699188,
0.2478924394,
-0.5998016596,
0.1715028137,
-0.2896076739,
-0.1076941863,
-0.0214398988,
-0.0624916218,
0.0274148695,
-0.0474656932,
-0.0685558096,
0.151992619,
0.0771345571,
-0.1671152562,
-0.0906845629,
0.0034199415,
0.3101094067,
-0.3221139908,
0.1657060385,
0.2421717942,
0.1955044568,
-0.0940227062,
-0.1976960301,
-0.1558781862,
0.1051340252,
0.3647212386,
0.0791877508,
0.1687752157,
0.0262405798,
0.0895476192,
0.1797856688,
-0.2070019245,
0.2181633115,
0.0123555213,
0.2408843637,
0.0950062498,
-0.0293945745,
-0.3097334206,
0.4583242834,
0.2681970894,
0.2772993445,
0.2424815446,
-0.4937293231,
-0.0740423277,
-0.0656516552,
-0.0718549043,
0.3221568167,
0.050078582,
-0.1195638403,
0.1201549098,
0.1963380426,
0.009703177,
0.3097597659,
0.1743883491,
0.4940780401,
-0.0187084917,
0.1057225913,
-0.0806348994,
-0.0995870829,
-0.1468482763,
0.0500457771,
0.507763803,
-0.1753805876,
-0.0157852508,
-0.1864269525,
-0.4006139934,
-0.2753978968,
0.1643144488,
-0.464242667,
-0.1369271427,
-0.384930104,
0.324758172,
0.0695632696,
0.2584399283,
0.4160760045,
-0.0507141761,
0.3513424397,
-0.1406715214,
0.0687717423,
-0.230412364,
-0.1190754846,
-0.0627370998,
0.4409624636,
-0.2204724401,
0.0868327916,
0.1567280591,
-0.3195577264,
-0.2135091126,
-0.1457151026,
0.146989584,
-0.0808213428,
0.1255743355,
0.0596420467,
0.5084346533,
-0.1233983934,
-0.2323761433,
0.1721096337,
0.0899068639,
-0.058872208,
-0.0373006128,
0.0232935697,
0.1553850174,
0.0540890247,
-0.2931140363,
-0.1797574908,
-0.5081845522,
0.4014801979,
-0.0338908918,
0.1513631344,
0.3279176652,
0.1617627889,
0.1156152114,
-0.1213659048,
0.089448072,
-0.1621508747,
0.0160201583,
0.3207913637,
-0.1488260329,
-0.211434871,
-0.0290637761,
0.0627377853,
0.3063040078,
0.2301857173,
-0.6157576442,
-0.0209110267,
-0.1136819571,
-0.0108944923,
-0.0588510372,
0.286311388,
0.3913798928,
0.0789214522,
0.1078000814,
0.1375888139,
-0.1677573621,
0.0949070081,
-0.0409829654,
0.3248966634,
0.2200790942,
0.5234264135,
0.063726522,
0.8384485841,
0.3419641554,
0.187140435,
0.2288258374,
-0.0220659506,
0.0835646093,
0.0049700928,
-0.3481869698,
-0.0082088821,
-0.0368969217,
0.0619997904,
0.1661427766,
0.0087551922,
-0.3374429047,
-0.1676723063,
-0.3505286574,
0.0459903963,
-0.3771667182,
0.2698537111,
0.1751248091,
0.3674234748,
-0.0838354081,
-0.0824134573,
0.0231612474,
-0.3515779972,
0.1945329756,
0.2135324776,
-0.0175285209,
0.0211244822,
-0.1137083545,
-0.3958894312,
-0.6405463219,
0.1182040945,
-0.1544020027,
0.0629795864,
-0.1038482636,
0.0933977216,
0.0600523278,
0.158307448,
0.7553269863,
0.0059234402,
-0.3072927296,
-0.0461269282,
-0.2609418631,
-0.5063740611,
0.2137844414,
-0.1841569394,
0.3441571891,
0.1433893144,
0.5205534697,
-0.3616020083,
-0.0981341898,
0.3212879598,
0.3747146726,
-0.2170826793,
-0.204692319,
-0.1343318969,
-0.2551261783,
-0.6043949723,
0.0041028555,
0.0545694306,
0.1934489608,
0.4964525402,
0.2044561356,
0.0526690781,
-0.0508969165,
0.1371939182,
-0.0533755906,
0.3105923831,
0.1106538773,
0.1008514613,
0.1345039904,
-0.1580678374,
0.3764191866,
0.2939083576,
0.0716901049,
-0.379755348,
-0.0997852832,
0.0576215051,
0.2523964643,
-0.0627553761,
-0.0139148347,
0.0038341142,
0.0268993154,
0.1780953854,
-0.0997750536,
0.1322199404,
0.187568143,
0.0874078646,
-0.4511453807,
-0.4366311729,
0.3414417505,
0.1997043639,
0.1596940011,
0.3301561475,
-0.1424446404,
-0.5566367507,
0.3253409564,
0.3589582145,
0.9608319998,
-0.3551482558,
0.3937278986,
0.0962183401,
0.2117650509,
0.6567655802,
-0.4751399457,
0.35296157,
-0.3699404895,
-0.081575796,
0.0431751236,
-0.1330996454,
0.1379618049,
-0.0172324833,
-0.4360865951,
0.2891325355,
0.0913326368,
-0.0398276001,
-0.1840587556,
0.3883706033,
0.1124473065,
-0.48119995,
-0.1841866821,
0.0319510885,
-0.1269842386,
0.2439667881,
-0.0456033051,
-0.0274405442,
0.115675956,
-0.1150274202,
-0.3924034834,
-0.0374513194,
-0.4836361706,
0.3076910377,
-0.2517832816,
0.0015951523,
0.4047461748,
0.3928067386,
0.0101811597,
0.369848609,
-0.1340499073,
0.0105265826,
-0.3600814641,
-0.2767651975,
-0.185056746,
0.0676644444,
0.2424039692,
-0.1937426329,
-0.1071276665,
0.0763599202,
-0.0399470702,
-0.1982410252,
-0.2103526294,
-0.0318766981,
-0.1298190653,
-0.2045655698,
-0.2123932242,
0.0132842474,
-0.3584756553,
-0.0186202638,
0.0458631888,
0.0108469883,
-0.3378366232,
0.3286833167,
0.1423628628,
-0.4008429945,
0.0307401512,
0.4164580703,
0.3485439122,
0.1605853885,
0.7200255394,
0.323261708,
-0.2456385195,
-0.1618595421,
-0.0824731737,
-0.0253359526,
-0.3048343062,
0.2100924999,
0.0837552622,
0.1060535014,
-0.3066003621,
-0.0003774762,
0.1285433918,
0.0452356935,
0.0363890566,
-0.3043984175,
-0.5601997972,
0.2082496285,
-0.0104781119,
0.0364991426,
0.2368942797,
0.0221907832,
0.0295316279,
0.2480151206,
-0.156630531,
0.0200542696,
-0.1691418737,
0.2507919371,
0.2438192368,
-0.1116869524,
0.1032437086,
-0.0129490774,
-0.0043390519,
0.1593104899,
-0.0150238331,
-0.1269527674,
0.0454817601,
0.1532625705,
-0.0268511418,
-0.2777855098,
-0.0963580906,
-0.543037951,
-0.0158970244,
-0.303994,
0.011127837,
0.0212790836,
-0.1362703741,
0.110977523,
0.2019028813,
-0.273165822,
-0.0630257353,
0.2792617679,
-0.0221644267,
-0.0507524982,
0.1658708006,
0.2017491758,
0.093798995,
-0.1570738405,
-0.5362299681,
0.0558344983,
-0.0843360648,
-0.0266278628,
0.1019935533,
-0.1142850742,
-0.3993760049,
0.3194215596,
0.0904667899,
0.1510136276,
-0.1423276216,
0.2068411559,
0.2004514337,
0.0089689372,
-0.1937268972,
-0.0418561623,
0.330198884,
-0.2920087278,
-0.0505977869,
0.3277902603,
-0.1311672479,
0.0453455485,
-0.0299583618,
0.131158188,
0.3588021398,
-0.0734307617,
0.3226176202,
0.0061582257,
-0.0834841952,
-0.0167206824,
0.1939019263,
0.4057277441,
0.2587029934,
0.2043865174,
-0.1556383669,
0.0376388319,
-0.1058721393,
-0.2548226416,
0.0379431695,
-0.0836716592,
0.1414890736,
0.4189735055,
-0.1775915623,
0.1592200845,
-0.1939132512,
0.1780177057,
0.1845970601,
-0.2745579481,
0.0787767842,
0.2984714806,
-0.2116875499,
0.0464743972,
0.1499888301,
-0.1157813296,
-0.0657881498,
0.1417854428,
0.0771140009,
-0.6087389588,
0.4591957331,
0.1467867196,
-0.2206506431,
-0.1409263164,
0.2773649096,
0.4522538483,
0.0710783973,
-0.3053993285,
0.0575941615,
0.0269877221,
0.1365890056,
-0.3318276703,
0.3094460368,
0.4621621668,
0.2915848494,
-0.1956234574,
0.2535726428,
-0.1581638753,
-0.0126190586,
0.015425398,
-0.0236887503,
0.1164659038,
0.2165058404,
0.1130550504,
-0.0109330351,
-0.0586275011,
-0.1510954201,
0.1630201936,
0.1425204277,
-0.2279163748,
-0.3811418712,
-0.0991924331,
-0.1710889935,
-0.0140400529,
-0.0115698958,
-0.5901708007,
0.2826707363,
0.5286231637,
-0.1380670071,
0.0812101886,
0.0202329364,
0.0137295788,
-0.0319661349,
0.4846237004,
0.2030229568,
-0.0330805741,
-0.4814165831,
-0.3177812994,
-0.4150763154,
0.0241080243,
-0.3297815025,
0.0040631229,
-0.0754074827,
0.1153875366,
-0.0473553129,
0.0465940088,
0.0486276522,
-0.3642247021,
-0.0435177833,
0.1776142716,
-0.1560724527,
-0.2553374767,
-0.1273900121,
0.1787595302,
0.179388836,
-0.1981145144,
0.2631113529,
0.008783441,
-0.1398376077,
-0.3518666327,
0.1338684708,
0.0501968861,
0.1779215187,
0.1576357484,
0.0610057488,
0.3895050287,
0.0040852698,
-0.2422716767,
0.0258158147,
0.0002693438,
-0.0998136923,
0.1413407922,
0.1294570714,
0.3691282868,
-0.4097651839,
-0.3827790916,
-0.4327932894,
0.2897743881,
-0.0637848526,
-0.1197689697,
-0.0350802019,
-0.0376016907,
0.2526534796,
0.0688294843,
0.0636018366,
0.1586665064,
-0.0656889006,
-0.0003349491,
-0.4509043992,
-0.2615719438,
0.4598853588,
-0.4583000839,
-0.3856024146,
-0.0861000121,
-0.0950355083,
-0.1459356695,
-0.1394123882,
-0.7795309424,
0.0910597444,
0.2944115102,
0.095530577,
-0.4224689901,
0.0314958505,
-0.1058145165,
0.1201660037,
-0.1256256849,
0.3365495503,
-0.0900949836,
-0.4325063527,
0.1651303619,
-0.2077715397
]
|
https://github.com/huggingface/datasets/issues/1989 | Question/problem with dataset labels | It seems that I get parsing errors for various fields in my data. For example now I get this:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: https://www.netgalley.com/catalog/book/121872
``` | Hi, I'm using a dataset with two labels "nurse" and "not nurse". For whatever reason (that I don't understand), I get an error that I think comes from the datasets package (using csv). Everything works fine if the labels are "nurse" and "surgeon".
This is the trace I get:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: not nurse
```
Any ideas how to fix this? For now, I'll probably make them numeric. | 128 | Question/problem with dataset labels
Hi, I'm using a dataset with two labels "nurse" and "not nurse". For whatever reason (that I don't understand), I get an error that I think comes from the datasets package (using csv). Everything works fine if the labels are "nurse" and "surgeon".
This is the trace I get:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: not nurse
```
Any ideas how to fix this? For now, I'll probably make them numeric.
It seems that I get parsing errors for various fields in my data. For example now I get this:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: https://www.netgalley.com/catalog/book/121872
``` | [
0.1789148301,
-0.0573230609,
0.0051793531,
0.1343671232,
0.4113537371,
0.3502124548,
0.6419945955,
0.1637074798,
-0.1613179296,
0.0723498836,
0.1788543165,
0.0638753474,
-0.0607729964,
0.030525703,
-0.130230993,
-0.1193704456,
0.0890837312,
0.1734791994,
0.1816426963,
-0.04446676,
-0.2867425382,
-0.0398259945,
-0.156679973,
0.3662885129,
-0.297205627,
-0.3766838312,
0.2105430812,
-0.1205282882,
-0.1131331921,
-0.4633954763,
0.2718095183,
0.0861200094,
0.0026800728,
0.4034268558,
-0.0001030963,
0.058756806,
0.2967477739,
0.1559889019,
-0.3984076679,
-0.4321287572,
-0.1893597841,
-0.0234080795,
0.0345638432,
-0.3044767976,
0.0979833305,
-0.2846682072,
-0.0905410498,
-0.2756658196,
0.1046864912,
0.4386059344,
0.3266399205,
0.2373396754,
-0.2065001428,
-0.2293031216,
0.2311526835,
0.1250885725,
-0.0305089504,
0.0494753644,
-0.1344386041,
-0.0064582187,
0.4515704513,
0.460914433,
-0.2723956704,
0.2647709846,
0.1353648007,
0.1463635266,
0.1377247274,
-0.3910481632,
0.2763096094,
0.1961432248,
0.7452604771,
-0.2964998186,
-0.2943911254,
-0.1697053015,
0.1373766065,
-0.4167195559,
0.1813390404,
0.0700832456,
0.0063756779,
-0.0470148437,
-0.0331627578,
0.0610270314,
0.0807417855,
0.0881804153,
-0.0580748878,
0.0383284204,
-0.0986412764,
0.3288168907,
0.1125301495,
-0.0747507066,
0.2975301445,
-0.0059941988,
-0.0885701999,
0.0415449142,
-0.4325241745,
-0.0296084415,
-0.0368810184,
0.0516759381,
-0.1181376055,
-0.038740769,
0.0183869507,
-0.3749563098,
-0.1474356651,
0.3403449953,
0.2151344717,
0.2662764788,
0.2706995606,
0.5616863966,
0.0911723822,
-0.0761627182,
0.0308040548,
-0.0558074787,
-0.0015739995,
-0.4626432061,
0.2433210015,
0.0989312604,
0.2934703231,
-0.2398585975,
-0.5455213189,
0.1924421936,
-0.0281014666,
0.0940956026,
0.1541178226,
0.3049503565,
0.0221220106,
0.2065178901,
0.0793469325,
0.193608433,
-0.0091703041,
-0.1172054783,
-0.2069241703,
0.0976080745,
-0.2002791017,
-0.150106892,
0.0502697304,
-0.0720530748,
0.1124490499,
0.0640597194,
-0.0010049042,
-0.0760566816,
-0.0163034722,
-0.3864641488,
-0.012748206,
0.3588934243,
-0.1032633781,
0.420350641,
0.2520543337,
-0.4185501039,
0.0596993491,
0.1802113652,
-0.3688521683,
-0.0338135473,
-0.2121574432,
0.2862125635,
-0.1030441746,
-0.187981084,
-0.1200913265,
0.0468237363,
0.4193198979,
-0.2145542502,
0.1575898081,
-0.3543840945,
-0.3093222678,
-0.2777702212,
-0.0663038418,
0.2214321792,
-0.6722466946,
0.004389429,
-0.1475467384,
-0.0291129835,
0.0528065376,
0.2960194051,
-0.0810041502,
0.2062916607,
-0.0705919117,
0.0303497873,
0.1395514011,
-0.390691489,
-0.1104637682,
0.1035439447,
-0.1420033723,
-0.2442642748,
0.0704807937,
-0.0403395221,
0.0917313397,
-0.0916260481,
0.0582318865,
-0.1318774968,
-0.1996321678,
-0.0951335654,
-0.1437036842,
0.0538111962,
0.653909564,
0.0927327722,
-0.016800072,
0.0756470934,
-0.1002285406,
-0.3448368311,
-0.0425327159,
-0.1009554341,
0.2062197328,
0.2147901356,
0.0556404777,
0.2983597219,
0.1459521502,
-0.0644702911,
-0.4424965978,
0.1017011777,
-0.0400518253,
0.1395645589,
-0.145143196,
-0.1923438162,
-0.3297647238,
-0.0537859611,
-0.2079762369,
0.0183238313,
0.1633132249,
0.0683607757,
-0.2214221507,
-0.0204832722,
0.0140843056,
-0.075884521,
-0.0172140431,
0.1093305945,
-0.1040251106,
0.2656517625,
-0.0951162279,
0.1168940216,
-0.0267393459,
0.2531200051,
0.4326063693,
0.2400517166,
-0.0466214195,
0.367023617,
0.0848606005,
-0.3270231187,
0.0078404322,
-0.172658667,
0.0181292761,
-0.0838742778,
-0.0163266249,
0.1145247817,
0.2768396139,
-0.1085973084,
-0.0847191364,
0.1411861628,
-0.1772004515,
0.2762841582,
-0.1619747579,
0.2004606873,
0.2599629462,
-0.1093928516,
0.1251222938,
-0.2127112299,
0.2158810794,
-0.1297985166,
0.2343143374,
0.007142223,
-0.2617177367,
0.0677190349,
0.4220539927,
0.0015310015,
-0.0035239446,
0.009774005,
-0.1586676836,
0.1657464355,
-0.0130180242,
0.595718503,
0.352842629,
0.2059556991,
-0.1638759673,
-0.0582683198,
-0.2622588873,
-0.1369839609,
0.2124615908,
0.0951014385,
0.0298523773,
0.0572962686,
0.0261101257,
-0.0664390028,
-0.1765725613,
-0.2433390021,
0.2082311809,
0.3464297354,
-0.51031214,
-0.0029065625,
-0.3452727795,
-0.2318637222,
-0.3185971677,
-0.0987910777,
-0.2351591289,
-0.4590440094,
0.0904379636,
-0.0774915814,
-0.1903930455,
0.1450763792,
-0.0375638828,
0.0079173651,
-0.0910730362,
0.1520518214,
-0.0975839868,
-0.4702498615,
-0.2045714557,
0.1811123341,
0.0074800067,
0.0469533242,
0.4813601077,
-0.1431986392,
-0.1245555803,
-0.0785720423,
-0.2953169942,
0.0040264316,
-0.2730659246,
0.1366041899,
0.1816231608,
0.103859432,
0.0084615927,
-0.1486845016,
0.2930162251,
-0.0715196803,
0.0246398468,
0.1069570333,
0.0452330634,
-0.2920732796,
-0.2664697468,
-0.4750192463,
-0.4745301306,
-0.1774818748,
0.0669479594,
-0.075908266,
-0.0335285738,
0.5198025703,
0.0684420913,
0.0656812266,
0.0931449234,
0.023252707,
-0.3467434645,
-0.0935407355,
0.2490479201,
-0.179091692,
-0.210245356,
0.1960575432,
0.1093095243,
0.1175282896,
-0.20393309,
-0.3139223158,
0.0728625953,
-0.0576012135,
0.058944717,
0.0813103691,
0.1948962212,
0.2138828039,
0.2671021223,
-0.1480035037,
-0.3270511925,
-0.3317118883,
0.1381734908,
-0.0719676912,
0.1306350976,
-0.1062558591,
0.294085592,
-0.152083829,
0.326508671,
0.0217723008,
-0.2126558274,
0.191888988,
-0.0478476658,
0.5207321048,
0.068380706,
-0.4193751216,
0.0658852011,
-0.1191071793,
0.0269460715,
0.1760428697,
-0.3196389079,
0.0379566401,
-0.1479681134,
0.3946042061,
-0.2478049397,
-0.0996567011,
-0.1006427184,
-0.1501683444,
0.0405269749,
0.1169003174,
-0.0409751162,
-0.2084082365,
-0.1915850341,
-0.2512801886,
0.0578238554,
-0.0954614133,
-0.087551482,
-0.533575058,
-0.2334993929,
-0.1649958491,
0.2940982878,
0.1574513167,
0.3346639276,
-0.1785171777,
0.2454715222,
0.0477584898,
0.2458998859,
0.6149811745,
-0.4832390547,
0.154702425,
0.2263719141,
0.3931272328,
-0.1780015528,
-0.1272553653,
-0.1692574769,
0.2947262228,
-0.2957772911,
0.0259887744,
-0.1555344164,
-0.0910770446,
0.2631006241,
0.1554057747,
-0.1223803461,
-0.3149805069,
-0.2309516966,
-0.1793929189,
-0.1272854507,
-0.0533969514,
0.0635773465,
0.1866380721,
-0.3784870207,
0.0986478776,
0.1408236176,
0.0735073909,
0.2715045512,
0.1186348274,
0.2232429981,
0.0302767064,
0.197361365,
0.317402184,
0.2587257028,
0.5099822879,
0.5483981967,
-0.3618466854,
-0.5113294125,
-0.1194789112,
-0.1147691086,
0.2007077336,
0.0650010705,
-0.1422151178,
0.1660957336,
-0.0506398827,
0.185458824,
0.0961978808,
0.0223078672,
0.4922952354,
0.1605764627,
-0.4898479283,
-0.5388931632,
0.2713592649,
0.0392414108,
-0.0478406474,
0.1281552166,
0.0797321871,
-0.3407573402,
0.2059525549,
-0.1559936553,
0.6908729672,
0.0837977454,
-0.0510304905,
-0.0170310941,
-0.2261731625,
0.7132234573,
-0.1187111512,
0.0007714578,
-0.3614293039,
-0.3074660599,
-0.0622299947,
-0.0221186746,
0.0234751571,
0.4506933987,
-0.3085790277,
0.3788379431,
-0.3285133839,
0.2046867758,
0.028750021,
-0.0740614757,
0.2494399399,
-0.1323137879,
-0.0824863017,
0.2010339946,
-0.1576190293,
-0.1343754977,
-0.1674345434,
0.0419054106,
-0.2318485528,
-0.2680606246,
-0.2262603641,
0.08226078,
-0.2538248897,
0.1415783167,
-0.0856540874,
-0.0167195015,
0.2168119252,
0.2025102079,
0.4863218665,
0.0789240599,
-0.1482862979,
0.1838956922,
0.0421487242,
0.1370235533,
0.0343489051,
0.0537468866,
0.4150622785,
0.1160496846,
-0.2699809074,
0.1285305768,
-0.0795674771,
0.1759498864,
-0.0870310813,
0.0838943273,
-0.0983974338,
-0.3653748333,
0.0326472521,
-0.0770386755,
0.2287106365,
-0.2537733614,
0.1773460358,
0.0519831851,
-0.0466800705,
0.2120788842,
-0.2636915445,
-0.1465975195,
-0.0457466282,
0.1892329752,
0.0259641036,
0.1933565587,
0.2472447455,
0.0259730872,
-0.3513919413,
-0.2565112412,
0.038952101,
0.0239994247,
-0.6778352261,
0.2459824234,
0.2533547282,
0.0759458542,
-0.057189174,
0.2232685387,
-0.0158301294,
0.0710849985,
0.0610140152,
-0.5274376869,
-0.2186500877,
-0.00467043,
-0.1847544909,
0.1169297621,
0.2478654385,
0.4110050797,
0.1337576956,
0.0714501962,
-0.3641543388,
-0.020954119,
-0.172394067,
0.2980129123,
0.1525106579,
0.0199611541,
0.2820312381,
-0.1788062751,
0.218216911,
0.0007437372,
0.007750046,
-0.2498184741,
-0.1872092783,
0.1124106348,
0.1352093369,
-0.1972157359,
-0.0634114593,
-0.1643812805,
0.0578369349,
-0.0719840601,
-0.0172511172,
0.3105739653,
0.0032078198,
0.3735309839,
-0.1509219557,
0.2327846885,
-0.0463298075,
0.2182779461,
0.0127172945,
-0.114001587,
0.0742451549,
0.1729656607,
-0.1127646267,
0.0194780901,
-0.1750794947,
0.0847947747,
-0.1170051619,
-0.11792963,
0.1342932731,
-0.568980515,
0.2959564924,
0.193638131,
0.449362725,
0.2781369686,
-0.1868256927,
-0.0707662776,
0.3826093972,
0.307926774,
-0.3656698763,
-0.1683599651,
0.1630016863,
0.1655610651,
0.0003906599,
0.0756075159,
0.060725417,
-0.0310598556,
0.0448926538,
0.0080858618,
0.4554387927,
-0.4376546144,
0.2349053174,
0.5269825459,
-0.0619708523,
0.0999840051,
0.4967942238,
0.1731765717,
0.0635329187,
0.5881978869,
0.0346502028,
0.1773207486,
0.1874702275,
-0.1379599422,
-0.0754204541,
-0.172217235,
0.1809182614,
0.0969726592,
0.0188562367,
-0.0428314172,
-0.0954477713,
0.3011343777,
-0.3839455247,
0.0041103126,
-0.0057690036,
0.1111971214,
-0.2255034149,
-0.1060157418,
-0.1562631726,
-0.0987095013,
-0.1664459705,
-0.1973160356,
0.0140280882,
-0.1700504124,
0.3556354642,
-0.0742809027,
-0.1959641278,
-0.4565812349,
-0.186778456,
0.305419594,
0.065081425,
-0.2473097742,
0.0163199063,
0.2487559468,
-0.1455008835,
0.3213778436,
0.086239703,
0.6039388776,
0.1713752747,
0.0897912681,
-0.3410916328,
-0.0972951949,
-0.088580206,
0.1516636312,
0.215568006,
0.0459204242,
-0.0368339717,
0.3922656178,
0.2018781453,
-0.1707493216,
0.3085803986,
0.3357422054,
0.2227042168,
-0.170619756,
-0.0630670115,
-0.1339656562,
-0.2573547959,
-0.3266397119,
0.1013188735,
-0.2697949111,
0.1159574091,
0.4622558057,
-0.0415135659,
0.0840901211,
-0.2942293584,
0.1462800503,
0.1317104101,
0.3118901849,
0.2739442885,
-0.0476244502,
-0.3456508517,
0.1615712494,
-0.5773795247,
0.129460454,
-0.1186997294,
-0.1146923974,
-0.0334540457,
-0.1582231671,
0.1593217105,
0.020614991,
0.3829501867,
0.1888182312,
-0.0159400646,
-0.1086774766,
-0.1195141748,
-0.1931985319,
-0.0825988874,
-0.1219008714,
0.2010165602,
-0.3226899803,
-0.0618356615,
-0.1457770467,
0.038975928,
-0.3498083949,
0.0937143192,
0.1062969789,
-0.0201894138,
0.3603177369,
0.1459479332,
0.7699726224,
0.048815161,
0.1150308847,
-0.0913875476,
-0.353649199,
-0.4042430222,
0.3688688576,
0.3255911171,
0.2565357983,
-0.1063772365,
0.0097641386,
-0.1644826233,
0.0945067257,
-0.04354655,
0.3211485744,
-0.2544759512,
0.2145047933,
-0.0240108576,
0.0171401687,
0.0991312191,
-0.0556259044,
-0.0026464916,
0.3410544395,
-0.2067562938,
-0.6047096252,
0.6198091507,
-0.212931633,
-0.2395552248,
0.1537303925,
0.1545257866,
0.2213794589,
-0.2187473923,
-0.5100530386,
0.0247500949,
0.3773825169,
0.0190039705,
-0.4423446655,
-0.0037064492,
-0.1927573532,
0.051444225,
0.113560237,
0.3062537014,
0.1166288406,
-0.1932859123,
0.1991046965,
-0.2543466091
]
|
https://github.com/huggingface/datasets/issues/1989 | Question/problem with dataset labels | Not sure if this helps, this is how I load my files (as in the sample scripts on transformers):
```
if data_args.train_file.endswith(".csv"):
# Loading a dataset from local csv files
datasets = load_dataset("csv", data_files=data_files)
``` | Hi, I'm using a dataset with two labels "nurse" and "not nurse". For whatever reason (that I don't understand), I get an error that I think comes from the datasets package (using csv). Everything works fine if the labels are "nurse" and "surgeon".
This is the trace I get:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: not nurse
```
Any ideas how to fix this? For now, I'll probably make them numeric. | 35 | Question/problem with dataset labels
Hi, I'm using a dataset with two labels "nurse" and "not nurse". For whatever reason (that I don't understand), I get an error that I think comes from the datasets package (using csv). Everything works fine if the labels are "nurse" and "surgeon".
This is the trace I get:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: not nurse
```
Any ideas how to fix this? For now, I'll probably make them numeric.
Not sure if this helps, this is how I load my files (as in the sample scripts on transformers):
```
if data_args.train_file.endswith(".csv"):
# Loading a dataset from local csv files
datasets = load_dataset("csv", data_files=data_files)
``` | [
0.1789148301,
-0.0573230609,
0.0051793531,
0.1343671232,
0.4113537371,
0.3502124548,
0.6419945955,
0.1637074798,
-0.1613179296,
0.0723498836,
0.1788543165,
0.0638753474,
-0.0607729964,
0.030525703,
-0.130230993,
-0.1193704456,
0.0890837312,
0.1734791994,
0.1816426963,
-0.04446676,
-0.2867425382,
-0.0398259945,
-0.156679973,
0.3662885129,
-0.297205627,
-0.3766838312,
0.2105430812,
-0.1205282882,
-0.1131331921,
-0.4633954763,
0.2718095183,
0.0861200094,
0.0026800728,
0.4034268558,
-0.0001030963,
0.058756806,
0.2967477739,
0.1559889019,
-0.3984076679,
-0.4321287572,
-0.1893597841,
-0.0234080795,
0.0345638432,
-0.3044767976,
0.0979833305,
-0.2846682072,
-0.0905410498,
-0.2756658196,
0.1046864912,
0.4386059344,
0.3266399205,
0.2373396754,
-0.2065001428,
-0.2293031216,
0.2311526835,
0.1250885725,
-0.0305089504,
0.0494753644,
-0.1344386041,
-0.0064582187,
0.4515704513,
0.460914433,
-0.2723956704,
0.2647709846,
0.1353648007,
0.1463635266,
0.1377247274,
-0.3910481632,
0.2763096094,
0.1961432248,
0.7452604771,
-0.2964998186,
-0.2943911254,
-0.1697053015,
0.1373766065,
-0.4167195559,
0.1813390404,
0.0700832456,
0.0063756779,
-0.0470148437,
-0.0331627578,
0.0610270314,
0.0807417855,
0.0881804153,
-0.0580748878,
0.0383284204,
-0.0986412764,
0.3288168907,
0.1125301495,
-0.0747507066,
0.2975301445,
-0.0059941988,
-0.0885701999,
0.0415449142,
-0.4325241745,
-0.0296084415,
-0.0368810184,
0.0516759381,
-0.1181376055,
-0.038740769,
0.0183869507,
-0.3749563098,
-0.1474356651,
0.3403449953,
0.2151344717,
0.2662764788,
0.2706995606,
0.5616863966,
0.0911723822,
-0.0761627182,
0.0308040548,
-0.0558074787,
-0.0015739995,
-0.4626432061,
0.2433210015,
0.0989312604,
0.2934703231,
-0.2398585975,
-0.5455213189,
0.1924421936,
-0.0281014666,
0.0940956026,
0.1541178226,
0.3049503565,
0.0221220106,
0.2065178901,
0.0793469325,
0.193608433,
-0.0091703041,
-0.1172054783,
-0.2069241703,
0.0976080745,
-0.2002791017,
-0.150106892,
0.0502697304,
-0.0720530748,
0.1124490499,
0.0640597194,
-0.0010049042,
-0.0760566816,
-0.0163034722,
-0.3864641488,
-0.012748206,
0.3588934243,
-0.1032633781,
0.420350641,
0.2520543337,
-0.4185501039,
0.0596993491,
0.1802113652,
-0.3688521683,
-0.0338135473,
-0.2121574432,
0.2862125635,
-0.1030441746,
-0.187981084,
-0.1200913265,
0.0468237363,
0.4193198979,
-0.2145542502,
0.1575898081,
-0.3543840945,
-0.3093222678,
-0.2777702212,
-0.0663038418,
0.2214321792,
-0.6722466946,
0.004389429,
-0.1475467384,
-0.0291129835,
0.0528065376,
0.2960194051,
-0.0810041502,
0.2062916607,
-0.0705919117,
0.0303497873,
0.1395514011,
-0.390691489,
-0.1104637682,
0.1035439447,
-0.1420033723,
-0.2442642748,
0.0704807937,
-0.0403395221,
0.0917313397,
-0.0916260481,
0.0582318865,
-0.1318774968,
-0.1996321678,
-0.0951335654,
-0.1437036842,
0.0538111962,
0.653909564,
0.0927327722,
-0.016800072,
0.0756470934,
-0.1002285406,
-0.3448368311,
-0.0425327159,
-0.1009554341,
0.2062197328,
0.2147901356,
0.0556404777,
0.2983597219,
0.1459521502,
-0.0644702911,
-0.4424965978,
0.1017011777,
-0.0400518253,
0.1395645589,
-0.145143196,
-0.1923438162,
-0.3297647238,
-0.0537859611,
-0.2079762369,
0.0183238313,
0.1633132249,
0.0683607757,
-0.2214221507,
-0.0204832722,
0.0140843056,
-0.075884521,
-0.0172140431,
0.1093305945,
-0.1040251106,
0.2656517625,
-0.0951162279,
0.1168940216,
-0.0267393459,
0.2531200051,
0.4326063693,
0.2400517166,
-0.0466214195,
0.367023617,
0.0848606005,
-0.3270231187,
0.0078404322,
-0.172658667,
0.0181292761,
-0.0838742778,
-0.0163266249,
0.1145247817,
0.2768396139,
-0.1085973084,
-0.0847191364,
0.1411861628,
-0.1772004515,
0.2762841582,
-0.1619747579,
0.2004606873,
0.2599629462,
-0.1093928516,
0.1251222938,
-0.2127112299,
0.2158810794,
-0.1297985166,
0.2343143374,
0.007142223,
-0.2617177367,
0.0677190349,
0.4220539927,
0.0015310015,
-0.0035239446,
0.009774005,
-0.1586676836,
0.1657464355,
-0.0130180242,
0.595718503,
0.352842629,
0.2059556991,
-0.1638759673,
-0.0582683198,
-0.2622588873,
-0.1369839609,
0.2124615908,
0.0951014385,
0.0298523773,
0.0572962686,
0.0261101257,
-0.0664390028,
-0.1765725613,
-0.2433390021,
0.2082311809,
0.3464297354,
-0.51031214,
-0.0029065625,
-0.3452727795,
-0.2318637222,
-0.3185971677,
-0.0987910777,
-0.2351591289,
-0.4590440094,
0.0904379636,
-0.0774915814,
-0.1903930455,
0.1450763792,
-0.0375638828,
0.0079173651,
-0.0910730362,
0.1520518214,
-0.0975839868,
-0.4702498615,
-0.2045714557,
0.1811123341,
0.0074800067,
0.0469533242,
0.4813601077,
-0.1431986392,
-0.1245555803,
-0.0785720423,
-0.2953169942,
0.0040264316,
-0.2730659246,
0.1366041899,
0.1816231608,
0.103859432,
0.0084615927,
-0.1486845016,
0.2930162251,
-0.0715196803,
0.0246398468,
0.1069570333,
0.0452330634,
-0.2920732796,
-0.2664697468,
-0.4750192463,
-0.4745301306,
-0.1774818748,
0.0669479594,
-0.075908266,
-0.0335285738,
0.5198025703,
0.0684420913,
0.0656812266,
0.0931449234,
0.023252707,
-0.3467434645,
-0.0935407355,
0.2490479201,
-0.179091692,
-0.210245356,
0.1960575432,
0.1093095243,
0.1175282896,
-0.20393309,
-0.3139223158,
0.0728625953,
-0.0576012135,
0.058944717,
0.0813103691,
0.1948962212,
0.2138828039,
0.2671021223,
-0.1480035037,
-0.3270511925,
-0.3317118883,
0.1381734908,
-0.0719676912,
0.1306350976,
-0.1062558591,
0.294085592,
-0.152083829,
0.326508671,
0.0217723008,
-0.2126558274,
0.191888988,
-0.0478476658,
0.5207321048,
0.068380706,
-0.4193751216,
0.0658852011,
-0.1191071793,
0.0269460715,
0.1760428697,
-0.3196389079,
0.0379566401,
-0.1479681134,
0.3946042061,
-0.2478049397,
-0.0996567011,
-0.1006427184,
-0.1501683444,
0.0405269749,
0.1169003174,
-0.0409751162,
-0.2084082365,
-0.1915850341,
-0.2512801886,
0.0578238554,
-0.0954614133,
-0.087551482,
-0.533575058,
-0.2334993929,
-0.1649958491,
0.2940982878,
0.1574513167,
0.3346639276,
-0.1785171777,
0.2454715222,
0.0477584898,
0.2458998859,
0.6149811745,
-0.4832390547,
0.154702425,
0.2263719141,
0.3931272328,
-0.1780015528,
-0.1272553653,
-0.1692574769,
0.2947262228,
-0.2957772911,
0.0259887744,
-0.1555344164,
-0.0910770446,
0.2631006241,
0.1554057747,
-0.1223803461,
-0.3149805069,
-0.2309516966,
-0.1793929189,
-0.1272854507,
-0.0533969514,
0.0635773465,
0.1866380721,
-0.3784870207,
0.0986478776,
0.1408236176,
0.0735073909,
0.2715045512,
0.1186348274,
0.2232429981,
0.0302767064,
0.197361365,
0.317402184,
0.2587257028,
0.5099822879,
0.5483981967,
-0.3618466854,
-0.5113294125,
-0.1194789112,
-0.1147691086,
0.2007077336,
0.0650010705,
-0.1422151178,
0.1660957336,
-0.0506398827,
0.185458824,
0.0961978808,
0.0223078672,
0.4922952354,
0.1605764627,
-0.4898479283,
-0.5388931632,
0.2713592649,
0.0392414108,
-0.0478406474,
0.1281552166,
0.0797321871,
-0.3407573402,
0.2059525549,
-0.1559936553,
0.6908729672,
0.0837977454,
-0.0510304905,
-0.0170310941,
-0.2261731625,
0.7132234573,
-0.1187111512,
0.0007714578,
-0.3614293039,
-0.3074660599,
-0.0622299947,
-0.0221186746,
0.0234751571,
0.4506933987,
-0.3085790277,
0.3788379431,
-0.3285133839,
0.2046867758,
0.028750021,
-0.0740614757,
0.2494399399,
-0.1323137879,
-0.0824863017,
0.2010339946,
-0.1576190293,
-0.1343754977,
-0.1674345434,
0.0419054106,
-0.2318485528,
-0.2680606246,
-0.2262603641,
0.08226078,
-0.2538248897,
0.1415783167,
-0.0856540874,
-0.0167195015,
0.2168119252,
0.2025102079,
0.4863218665,
0.0789240599,
-0.1482862979,
0.1838956922,
0.0421487242,
0.1370235533,
0.0343489051,
0.0537468866,
0.4150622785,
0.1160496846,
-0.2699809074,
0.1285305768,
-0.0795674771,
0.1759498864,
-0.0870310813,
0.0838943273,
-0.0983974338,
-0.3653748333,
0.0326472521,
-0.0770386755,
0.2287106365,
-0.2537733614,
0.1773460358,
0.0519831851,
-0.0466800705,
0.2120788842,
-0.2636915445,
-0.1465975195,
-0.0457466282,
0.1892329752,
0.0259641036,
0.1933565587,
0.2472447455,
0.0259730872,
-0.3513919413,
-0.2565112412,
0.038952101,
0.0239994247,
-0.6778352261,
0.2459824234,
0.2533547282,
0.0759458542,
-0.057189174,
0.2232685387,
-0.0158301294,
0.0710849985,
0.0610140152,
-0.5274376869,
-0.2186500877,
-0.00467043,
-0.1847544909,
0.1169297621,
0.2478654385,
0.4110050797,
0.1337576956,
0.0714501962,
-0.3641543388,
-0.020954119,
-0.172394067,
0.2980129123,
0.1525106579,
0.0199611541,
0.2820312381,
-0.1788062751,
0.218216911,
0.0007437372,
0.007750046,
-0.2498184741,
-0.1872092783,
0.1124106348,
0.1352093369,
-0.1972157359,
-0.0634114593,
-0.1643812805,
0.0578369349,
-0.0719840601,
-0.0172511172,
0.3105739653,
0.0032078198,
0.3735309839,
-0.1509219557,
0.2327846885,
-0.0463298075,
0.2182779461,
0.0127172945,
-0.114001587,
0.0742451549,
0.1729656607,
-0.1127646267,
0.0194780901,
-0.1750794947,
0.0847947747,
-0.1170051619,
-0.11792963,
0.1342932731,
-0.568980515,
0.2959564924,
0.193638131,
0.449362725,
0.2781369686,
-0.1868256927,
-0.0707662776,
0.3826093972,
0.307926774,
-0.3656698763,
-0.1683599651,
0.1630016863,
0.1655610651,
0.0003906599,
0.0756075159,
0.060725417,
-0.0310598556,
0.0448926538,
0.0080858618,
0.4554387927,
-0.4376546144,
0.2349053174,
0.5269825459,
-0.0619708523,
0.0999840051,
0.4967942238,
0.1731765717,
0.0635329187,
0.5881978869,
0.0346502028,
0.1773207486,
0.1874702275,
-0.1379599422,
-0.0754204541,
-0.172217235,
0.1809182614,
0.0969726592,
0.0188562367,
-0.0428314172,
-0.0954477713,
0.3011343777,
-0.3839455247,
0.0041103126,
-0.0057690036,
0.1111971214,
-0.2255034149,
-0.1060157418,
-0.1562631726,
-0.0987095013,
-0.1664459705,
-0.1973160356,
0.0140280882,
-0.1700504124,
0.3556354642,
-0.0742809027,
-0.1959641278,
-0.4565812349,
-0.186778456,
0.305419594,
0.065081425,
-0.2473097742,
0.0163199063,
0.2487559468,
-0.1455008835,
0.3213778436,
0.086239703,
0.6039388776,
0.1713752747,
0.0897912681,
-0.3410916328,
-0.0972951949,
-0.088580206,
0.1516636312,
0.215568006,
0.0459204242,
-0.0368339717,
0.3922656178,
0.2018781453,
-0.1707493216,
0.3085803986,
0.3357422054,
0.2227042168,
-0.170619756,
-0.0630670115,
-0.1339656562,
-0.2573547959,
-0.3266397119,
0.1013188735,
-0.2697949111,
0.1159574091,
0.4622558057,
-0.0415135659,
0.0840901211,
-0.2942293584,
0.1462800503,
0.1317104101,
0.3118901849,
0.2739442885,
-0.0476244502,
-0.3456508517,
0.1615712494,
-0.5773795247,
0.129460454,
-0.1186997294,
-0.1146923974,
-0.0334540457,
-0.1582231671,
0.1593217105,
0.020614991,
0.3829501867,
0.1888182312,
-0.0159400646,
-0.1086774766,
-0.1195141748,
-0.1931985319,
-0.0825988874,
-0.1219008714,
0.2010165602,
-0.3226899803,
-0.0618356615,
-0.1457770467,
0.038975928,
-0.3498083949,
0.0937143192,
0.1062969789,
-0.0201894138,
0.3603177369,
0.1459479332,
0.7699726224,
0.048815161,
0.1150308847,
-0.0913875476,
-0.353649199,
-0.4042430222,
0.3688688576,
0.3255911171,
0.2565357983,
-0.1063772365,
0.0097641386,
-0.1644826233,
0.0945067257,
-0.04354655,
0.3211485744,
-0.2544759512,
0.2145047933,
-0.0240108576,
0.0171401687,
0.0991312191,
-0.0556259044,
-0.0026464916,
0.3410544395,
-0.2067562938,
-0.6047096252,
0.6198091507,
-0.212931633,
-0.2395552248,
0.1537303925,
0.1545257866,
0.2213794589,
-0.2187473923,
-0.5100530386,
0.0247500949,
0.3773825169,
0.0190039705,
-0.4423446655,
-0.0037064492,
-0.1927573532,
0.051444225,
0.113560237,
0.3062537014,
0.1166288406,
-0.1932859123,
0.1991046965,
-0.2543466091
]
|
https://github.com/huggingface/datasets/issues/1989 | Question/problem with dataset labels | Since this worked out of the box in a few examples before, I wonder if it's some quoting issue or something else. | Hi, I'm using a dataset with two labels "nurse" and "not nurse". For whatever reason (that I don't understand), I get an error that I think comes from the datasets package (using csv). Everything works fine if the labels are "nurse" and "surgeon".
This is the trace I get:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: not nurse
```
Any ideas how to fix this? For now, I'll probably make them numeric. | 22 | Question/problem with dataset labels
Hi, I'm using a dataset with two labels "nurse" and "not nurse". For whatever reason (that I don't understand), I get an error that I think comes from the datasets package (using csv). Everything works fine if the labels are "nurse" and "surgeon".
This is the trace I get:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: not nurse
```
Any ideas how to fix this? For now, I'll probably make them numeric.
Since this worked out of the box in a few examples before, I wonder if it's some quoting issue or something else. | [
0.1789148301,
-0.0573230609,
0.0051793531,
0.1343671232,
0.4113537371,
0.3502124548,
0.6419945955,
0.1637074798,
-0.1613179296,
0.0723498836,
0.1788543165,
0.0638753474,
-0.0607729964,
0.030525703,
-0.130230993,
-0.1193704456,
0.0890837312,
0.1734791994,
0.1816426963,
-0.04446676,
-0.2867425382,
-0.0398259945,
-0.156679973,
0.3662885129,
-0.297205627,
-0.3766838312,
0.2105430812,
-0.1205282882,
-0.1131331921,
-0.4633954763,
0.2718095183,
0.0861200094,
0.0026800728,
0.4034268558,
-0.0001030963,
0.058756806,
0.2967477739,
0.1559889019,
-0.3984076679,
-0.4321287572,
-0.1893597841,
-0.0234080795,
0.0345638432,
-0.3044767976,
0.0979833305,
-0.2846682072,
-0.0905410498,
-0.2756658196,
0.1046864912,
0.4386059344,
0.3266399205,
0.2373396754,
-0.2065001428,
-0.2293031216,
0.2311526835,
0.1250885725,
-0.0305089504,
0.0494753644,
-0.1344386041,
-0.0064582187,
0.4515704513,
0.460914433,
-0.2723956704,
0.2647709846,
0.1353648007,
0.1463635266,
0.1377247274,
-0.3910481632,
0.2763096094,
0.1961432248,
0.7452604771,
-0.2964998186,
-0.2943911254,
-0.1697053015,
0.1373766065,
-0.4167195559,
0.1813390404,
0.0700832456,
0.0063756779,
-0.0470148437,
-0.0331627578,
0.0610270314,
0.0807417855,
0.0881804153,
-0.0580748878,
0.0383284204,
-0.0986412764,
0.3288168907,
0.1125301495,
-0.0747507066,
0.2975301445,
-0.0059941988,
-0.0885701999,
0.0415449142,
-0.4325241745,
-0.0296084415,
-0.0368810184,
0.0516759381,
-0.1181376055,
-0.038740769,
0.0183869507,
-0.3749563098,
-0.1474356651,
0.3403449953,
0.2151344717,
0.2662764788,
0.2706995606,
0.5616863966,
0.0911723822,
-0.0761627182,
0.0308040548,
-0.0558074787,
-0.0015739995,
-0.4626432061,
0.2433210015,
0.0989312604,
0.2934703231,
-0.2398585975,
-0.5455213189,
0.1924421936,
-0.0281014666,
0.0940956026,
0.1541178226,
0.3049503565,
0.0221220106,
0.2065178901,
0.0793469325,
0.193608433,
-0.0091703041,
-0.1172054783,
-0.2069241703,
0.0976080745,
-0.2002791017,
-0.150106892,
0.0502697304,
-0.0720530748,
0.1124490499,
0.0640597194,
-0.0010049042,
-0.0760566816,
-0.0163034722,
-0.3864641488,
-0.012748206,
0.3588934243,
-0.1032633781,
0.420350641,
0.2520543337,
-0.4185501039,
0.0596993491,
0.1802113652,
-0.3688521683,
-0.0338135473,
-0.2121574432,
0.2862125635,
-0.1030441746,
-0.187981084,
-0.1200913265,
0.0468237363,
0.4193198979,
-0.2145542502,
0.1575898081,
-0.3543840945,
-0.3093222678,
-0.2777702212,
-0.0663038418,
0.2214321792,
-0.6722466946,
0.004389429,
-0.1475467384,
-0.0291129835,
0.0528065376,
0.2960194051,
-0.0810041502,
0.2062916607,
-0.0705919117,
0.0303497873,
0.1395514011,
-0.390691489,
-0.1104637682,
0.1035439447,
-0.1420033723,
-0.2442642748,
0.0704807937,
-0.0403395221,
0.0917313397,
-0.0916260481,
0.0582318865,
-0.1318774968,
-0.1996321678,
-0.0951335654,
-0.1437036842,
0.0538111962,
0.653909564,
0.0927327722,
-0.016800072,
0.0756470934,
-0.1002285406,
-0.3448368311,
-0.0425327159,
-0.1009554341,
0.2062197328,
0.2147901356,
0.0556404777,
0.2983597219,
0.1459521502,
-0.0644702911,
-0.4424965978,
0.1017011777,
-0.0400518253,
0.1395645589,
-0.145143196,
-0.1923438162,
-0.3297647238,
-0.0537859611,
-0.2079762369,
0.0183238313,
0.1633132249,
0.0683607757,
-0.2214221507,
-0.0204832722,
0.0140843056,
-0.075884521,
-0.0172140431,
0.1093305945,
-0.1040251106,
0.2656517625,
-0.0951162279,
0.1168940216,
-0.0267393459,
0.2531200051,
0.4326063693,
0.2400517166,
-0.0466214195,
0.367023617,
0.0848606005,
-0.3270231187,
0.0078404322,
-0.172658667,
0.0181292761,
-0.0838742778,
-0.0163266249,
0.1145247817,
0.2768396139,
-0.1085973084,
-0.0847191364,
0.1411861628,
-0.1772004515,
0.2762841582,
-0.1619747579,
0.2004606873,
0.2599629462,
-0.1093928516,
0.1251222938,
-0.2127112299,
0.2158810794,
-0.1297985166,
0.2343143374,
0.007142223,
-0.2617177367,
0.0677190349,
0.4220539927,
0.0015310015,
-0.0035239446,
0.009774005,
-0.1586676836,
0.1657464355,
-0.0130180242,
0.595718503,
0.352842629,
0.2059556991,
-0.1638759673,
-0.0582683198,
-0.2622588873,
-0.1369839609,
0.2124615908,
0.0951014385,
0.0298523773,
0.0572962686,
0.0261101257,
-0.0664390028,
-0.1765725613,
-0.2433390021,
0.2082311809,
0.3464297354,
-0.51031214,
-0.0029065625,
-0.3452727795,
-0.2318637222,
-0.3185971677,
-0.0987910777,
-0.2351591289,
-0.4590440094,
0.0904379636,
-0.0774915814,
-0.1903930455,
0.1450763792,
-0.0375638828,
0.0079173651,
-0.0910730362,
0.1520518214,
-0.0975839868,
-0.4702498615,
-0.2045714557,
0.1811123341,
0.0074800067,
0.0469533242,
0.4813601077,
-0.1431986392,
-0.1245555803,
-0.0785720423,
-0.2953169942,
0.0040264316,
-0.2730659246,
0.1366041899,
0.1816231608,
0.103859432,
0.0084615927,
-0.1486845016,
0.2930162251,
-0.0715196803,
0.0246398468,
0.1069570333,
0.0452330634,
-0.2920732796,
-0.2664697468,
-0.4750192463,
-0.4745301306,
-0.1774818748,
0.0669479594,
-0.075908266,
-0.0335285738,
0.5198025703,
0.0684420913,
0.0656812266,
0.0931449234,
0.023252707,
-0.3467434645,
-0.0935407355,
0.2490479201,
-0.179091692,
-0.210245356,
0.1960575432,
0.1093095243,
0.1175282896,
-0.20393309,
-0.3139223158,
0.0728625953,
-0.0576012135,
0.058944717,
0.0813103691,
0.1948962212,
0.2138828039,
0.2671021223,
-0.1480035037,
-0.3270511925,
-0.3317118883,
0.1381734908,
-0.0719676912,
0.1306350976,
-0.1062558591,
0.294085592,
-0.152083829,
0.326508671,
0.0217723008,
-0.2126558274,
0.191888988,
-0.0478476658,
0.5207321048,
0.068380706,
-0.4193751216,
0.0658852011,
-0.1191071793,
0.0269460715,
0.1760428697,
-0.3196389079,
0.0379566401,
-0.1479681134,
0.3946042061,
-0.2478049397,
-0.0996567011,
-0.1006427184,
-0.1501683444,
0.0405269749,
0.1169003174,
-0.0409751162,
-0.2084082365,
-0.1915850341,
-0.2512801886,
0.0578238554,
-0.0954614133,
-0.087551482,
-0.533575058,
-0.2334993929,
-0.1649958491,
0.2940982878,
0.1574513167,
0.3346639276,
-0.1785171777,
0.2454715222,
0.0477584898,
0.2458998859,
0.6149811745,
-0.4832390547,
0.154702425,
0.2263719141,
0.3931272328,
-0.1780015528,
-0.1272553653,
-0.1692574769,
0.2947262228,
-0.2957772911,
0.0259887744,
-0.1555344164,
-0.0910770446,
0.2631006241,
0.1554057747,
-0.1223803461,
-0.3149805069,
-0.2309516966,
-0.1793929189,
-0.1272854507,
-0.0533969514,
0.0635773465,
0.1866380721,
-0.3784870207,
0.0986478776,
0.1408236176,
0.0735073909,
0.2715045512,
0.1186348274,
0.2232429981,
0.0302767064,
0.197361365,
0.317402184,
0.2587257028,
0.5099822879,
0.5483981967,
-0.3618466854,
-0.5113294125,
-0.1194789112,
-0.1147691086,
0.2007077336,
0.0650010705,
-0.1422151178,
0.1660957336,
-0.0506398827,
0.185458824,
0.0961978808,
0.0223078672,
0.4922952354,
0.1605764627,
-0.4898479283,
-0.5388931632,
0.2713592649,
0.0392414108,
-0.0478406474,
0.1281552166,
0.0797321871,
-0.3407573402,
0.2059525549,
-0.1559936553,
0.6908729672,
0.0837977454,
-0.0510304905,
-0.0170310941,
-0.2261731625,
0.7132234573,
-0.1187111512,
0.0007714578,
-0.3614293039,
-0.3074660599,
-0.0622299947,
-0.0221186746,
0.0234751571,
0.4506933987,
-0.3085790277,
0.3788379431,
-0.3285133839,
0.2046867758,
0.028750021,
-0.0740614757,
0.2494399399,
-0.1323137879,
-0.0824863017,
0.2010339946,
-0.1576190293,
-0.1343754977,
-0.1674345434,
0.0419054106,
-0.2318485528,
-0.2680606246,
-0.2262603641,
0.08226078,
-0.2538248897,
0.1415783167,
-0.0856540874,
-0.0167195015,
0.2168119252,
0.2025102079,
0.4863218665,
0.0789240599,
-0.1482862979,
0.1838956922,
0.0421487242,
0.1370235533,
0.0343489051,
0.0537468866,
0.4150622785,
0.1160496846,
-0.2699809074,
0.1285305768,
-0.0795674771,
0.1759498864,
-0.0870310813,
0.0838943273,
-0.0983974338,
-0.3653748333,
0.0326472521,
-0.0770386755,
0.2287106365,
-0.2537733614,
0.1773460358,
0.0519831851,
-0.0466800705,
0.2120788842,
-0.2636915445,
-0.1465975195,
-0.0457466282,
0.1892329752,
0.0259641036,
0.1933565587,
0.2472447455,
0.0259730872,
-0.3513919413,
-0.2565112412,
0.038952101,
0.0239994247,
-0.6778352261,
0.2459824234,
0.2533547282,
0.0759458542,
-0.057189174,
0.2232685387,
-0.0158301294,
0.0710849985,
0.0610140152,
-0.5274376869,
-0.2186500877,
-0.00467043,
-0.1847544909,
0.1169297621,
0.2478654385,
0.4110050797,
0.1337576956,
0.0714501962,
-0.3641543388,
-0.020954119,
-0.172394067,
0.2980129123,
0.1525106579,
0.0199611541,
0.2820312381,
-0.1788062751,
0.218216911,
0.0007437372,
0.007750046,
-0.2498184741,
-0.1872092783,
0.1124106348,
0.1352093369,
-0.1972157359,
-0.0634114593,
-0.1643812805,
0.0578369349,
-0.0719840601,
-0.0172511172,
0.3105739653,
0.0032078198,
0.3735309839,
-0.1509219557,
0.2327846885,
-0.0463298075,
0.2182779461,
0.0127172945,
-0.114001587,
0.0742451549,
0.1729656607,
-0.1127646267,
0.0194780901,
-0.1750794947,
0.0847947747,
-0.1170051619,
-0.11792963,
0.1342932731,
-0.568980515,
0.2959564924,
0.193638131,
0.449362725,
0.2781369686,
-0.1868256927,
-0.0707662776,
0.3826093972,
0.307926774,
-0.3656698763,
-0.1683599651,
0.1630016863,
0.1655610651,
0.0003906599,
0.0756075159,
0.060725417,
-0.0310598556,
0.0448926538,
0.0080858618,
0.4554387927,
-0.4376546144,
0.2349053174,
0.5269825459,
-0.0619708523,
0.0999840051,
0.4967942238,
0.1731765717,
0.0635329187,
0.5881978869,
0.0346502028,
0.1773207486,
0.1874702275,
-0.1379599422,
-0.0754204541,
-0.172217235,
0.1809182614,
0.0969726592,
0.0188562367,
-0.0428314172,
-0.0954477713,
0.3011343777,
-0.3839455247,
0.0041103126,
-0.0057690036,
0.1111971214,
-0.2255034149,
-0.1060157418,
-0.1562631726,
-0.0987095013,
-0.1664459705,
-0.1973160356,
0.0140280882,
-0.1700504124,
0.3556354642,
-0.0742809027,
-0.1959641278,
-0.4565812349,
-0.186778456,
0.305419594,
0.065081425,
-0.2473097742,
0.0163199063,
0.2487559468,
-0.1455008835,
0.3213778436,
0.086239703,
0.6039388776,
0.1713752747,
0.0897912681,
-0.3410916328,
-0.0972951949,
-0.088580206,
0.1516636312,
0.215568006,
0.0459204242,
-0.0368339717,
0.3922656178,
0.2018781453,
-0.1707493216,
0.3085803986,
0.3357422054,
0.2227042168,
-0.170619756,
-0.0630670115,
-0.1339656562,
-0.2573547959,
-0.3266397119,
0.1013188735,
-0.2697949111,
0.1159574091,
0.4622558057,
-0.0415135659,
0.0840901211,
-0.2942293584,
0.1462800503,
0.1317104101,
0.3118901849,
0.2739442885,
-0.0476244502,
-0.3456508517,
0.1615712494,
-0.5773795247,
0.129460454,
-0.1186997294,
-0.1146923974,
-0.0334540457,
-0.1582231671,
0.1593217105,
0.020614991,
0.3829501867,
0.1888182312,
-0.0159400646,
-0.1086774766,
-0.1195141748,
-0.1931985319,
-0.0825988874,
-0.1219008714,
0.2010165602,
-0.3226899803,
-0.0618356615,
-0.1457770467,
0.038975928,
-0.3498083949,
0.0937143192,
0.1062969789,
-0.0201894138,
0.3603177369,
0.1459479332,
0.7699726224,
0.048815161,
0.1150308847,
-0.0913875476,
-0.353649199,
-0.4042430222,
0.3688688576,
0.3255911171,
0.2565357983,
-0.1063772365,
0.0097641386,
-0.1644826233,
0.0945067257,
-0.04354655,
0.3211485744,
-0.2544759512,
0.2145047933,
-0.0240108576,
0.0171401687,
0.0991312191,
-0.0556259044,
-0.0026464916,
0.3410544395,
-0.2067562938,
-0.6047096252,
0.6198091507,
-0.212931633,
-0.2395552248,
0.1537303925,
0.1545257866,
0.2213794589,
-0.2187473923,
-0.5100530386,
0.0247500949,
0.3773825169,
0.0190039705,
-0.4423446655,
-0.0037064492,
-0.1927573532,
0.051444225,
0.113560237,
0.3062537014,
0.1166288406,
-0.1932859123,
0.1991046965,
-0.2543466091
]
|
https://github.com/huggingface/datasets/issues/1989 | Question/problem with dataset labels | Hi @ioana-blue,
Can you share a sample from your .csv? A dummy where you get this error will also help.
I tried this csv:
```csv
feature,label
1.2,not nurse
1.3,nurse
1.5,surgeon
```
and the following snippet:
```python
from datasets import load_dataset
d = load_dataset("csv",data_files=['test.csv'])
print(d)
print(d['train']['label'])
```
and this works perfectly fine for me:
```sh
DatasetDict({
train: Dataset({
features: ['feature', 'label'],
num_rows: 3
})
})
['not nurse', 'nurse', 'surgeon']
```
I'm sure your csv is more complicated than this one. But it is hard to tell where the issue might be without looking at a sample. | Hi, I'm using a dataset with two labels "nurse" and "not nurse". For whatever reason (that I don't understand), I get an error that I think comes from the datasets package (using csv). Everything works fine if the labels are "nurse" and "surgeon".
This is the trace I get:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: not nurse
```
Any ideas how to fix this? For now, I'll probably make them numeric. | 95 | Question/problem with dataset labels
Hi, I'm using a dataset with two labels "nurse" and "not nurse". For whatever reason (that I don't understand), I get an error that I think comes from the datasets package (using csv). Everything works fine if the labels are "nurse" and "surgeon".
This is the trace I get:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: not nurse
```
Any ideas how to fix this? For now, I'll probably make them numeric.
Hi @ioana-blue,
Can you share a sample from your .csv? A dummy where you get this error will also help.
I tried this csv:
```csv
feature,label
1.2,not nurse
1.3,nurse
1.5,surgeon
```
and the following snippet:
```python
from datasets import load_dataset
d = load_dataset("csv",data_files=['test.csv'])
print(d)
print(d['train']['label'])
```
and this works perfectly fine for me:
```sh
DatasetDict({
train: Dataset({
features: ['feature', 'label'],
num_rows: 3
})
})
['not nurse', 'nurse', 'surgeon']
```
I'm sure your csv is more complicated than this one. But it is hard to tell where the issue might be without looking at a sample. | [
0.1789148301,
-0.0573230609,
0.0051793531,
0.1343671232,
0.4113537371,
0.3502124548,
0.6419945955,
0.1637074798,
-0.1613179296,
0.0723498836,
0.1788543165,
0.0638753474,
-0.0607729964,
0.030525703,
-0.130230993,
-0.1193704456,
0.0890837312,
0.1734791994,
0.1816426963,
-0.04446676,
-0.2867425382,
-0.0398259945,
-0.156679973,
0.3662885129,
-0.297205627,
-0.3766838312,
0.2105430812,
-0.1205282882,
-0.1131331921,
-0.4633954763,
0.2718095183,
0.0861200094,
0.0026800728,
0.4034268558,
-0.0001030963,
0.058756806,
0.2967477739,
0.1559889019,
-0.3984076679,
-0.4321287572,
-0.1893597841,
-0.0234080795,
0.0345638432,
-0.3044767976,
0.0979833305,
-0.2846682072,
-0.0905410498,
-0.2756658196,
0.1046864912,
0.4386059344,
0.3266399205,
0.2373396754,
-0.2065001428,
-0.2293031216,
0.2311526835,
0.1250885725,
-0.0305089504,
0.0494753644,
-0.1344386041,
-0.0064582187,
0.4515704513,
0.460914433,
-0.2723956704,
0.2647709846,
0.1353648007,
0.1463635266,
0.1377247274,
-0.3910481632,
0.2763096094,
0.1961432248,
0.7452604771,
-0.2964998186,
-0.2943911254,
-0.1697053015,
0.1373766065,
-0.4167195559,
0.1813390404,
0.0700832456,
0.0063756779,
-0.0470148437,
-0.0331627578,
0.0610270314,
0.0807417855,
0.0881804153,
-0.0580748878,
0.0383284204,
-0.0986412764,
0.3288168907,
0.1125301495,
-0.0747507066,
0.2975301445,
-0.0059941988,
-0.0885701999,
0.0415449142,
-0.4325241745,
-0.0296084415,
-0.0368810184,
0.0516759381,
-0.1181376055,
-0.038740769,
0.0183869507,
-0.3749563098,
-0.1474356651,
0.3403449953,
0.2151344717,
0.2662764788,
0.2706995606,
0.5616863966,
0.0911723822,
-0.0761627182,
0.0308040548,
-0.0558074787,
-0.0015739995,
-0.4626432061,
0.2433210015,
0.0989312604,
0.2934703231,
-0.2398585975,
-0.5455213189,
0.1924421936,
-0.0281014666,
0.0940956026,
0.1541178226,
0.3049503565,
0.0221220106,
0.2065178901,
0.0793469325,
0.193608433,
-0.0091703041,
-0.1172054783,
-0.2069241703,
0.0976080745,
-0.2002791017,
-0.150106892,
0.0502697304,
-0.0720530748,
0.1124490499,
0.0640597194,
-0.0010049042,
-0.0760566816,
-0.0163034722,
-0.3864641488,
-0.012748206,
0.3588934243,
-0.1032633781,
0.420350641,
0.2520543337,
-0.4185501039,
0.0596993491,
0.1802113652,
-0.3688521683,
-0.0338135473,
-0.2121574432,
0.2862125635,
-0.1030441746,
-0.187981084,
-0.1200913265,
0.0468237363,
0.4193198979,
-0.2145542502,
0.1575898081,
-0.3543840945,
-0.3093222678,
-0.2777702212,
-0.0663038418,
0.2214321792,
-0.6722466946,
0.004389429,
-0.1475467384,
-0.0291129835,
0.0528065376,
0.2960194051,
-0.0810041502,
0.2062916607,
-0.0705919117,
0.0303497873,
0.1395514011,
-0.390691489,
-0.1104637682,
0.1035439447,
-0.1420033723,
-0.2442642748,
0.0704807937,
-0.0403395221,
0.0917313397,
-0.0916260481,
0.0582318865,
-0.1318774968,
-0.1996321678,
-0.0951335654,
-0.1437036842,
0.0538111962,
0.653909564,
0.0927327722,
-0.016800072,
0.0756470934,
-0.1002285406,
-0.3448368311,
-0.0425327159,
-0.1009554341,
0.2062197328,
0.2147901356,
0.0556404777,
0.2983597219,
0.1459521502,
-0.0644702911,
-0.4424965978,
0.1017011777,
-0.0400518253,
0.1395645589,
-0.145143196,
-0.1923438162,
-0.3297647238,
-0.0537859611,
-0.2079762369,
0.0183238313,
0.1633132249,
0.0683607757,
-0.2214221507,
-0.0204832722,
0.0140843056,
-0.075884521,
-0.0172140431,
0.1093305945,
-0.1040251106,
0.2656517625,
-0.0951162279,
0.1168940216,
-0.0267393459,
0.2531200051,
0.4326063693,
0.2400517166,
-0.0466214195,
0.367023617,
0.0848606005,
-0.3270231187,
0.0078404322,
-0.172658667,
0.0181292761,
-0.0838742778,
-0.0163266249,
0.1145247817,
0.2768396139,
-0.1085973084,
-0.0847191364,
0.1411861628,
-0.1772004515,
0.2762841582,
-0.1619747579,
0.2004606873,
0.2599629462,
-0.1093928516,
0.1251222938,
-0.2127112299,
0.2158810794,
-0.1297985166,
0.2343143374,
0.007142223,
-0.2617177367,
0.0677190349,
0.4220539927,
0.0015310015,
-0.0035239446,
0.009774005,
-0.1586676836,
0.1657464355,
-0.0130180242,
0.595718503,
0.352842629,
0.2059556991,
-0.1638759673,
-0.0582683198,
-0.2622588873,
-0.1369839609,
0.2124615908,
0.0951014385,
0.0298523773,
0.0572962686,
0.0261101257,
-0.0664390028,
-0.1765725613,
-0.2433390021,
0.2082311809,
0.3464297354,
-0.51031214,
-0.0029065625,
-0.3452727795,
-0.2318637222,
-0.3185971677,
-0.0987910777,
-0.2351591289,
-0.4590440094,
0.0904379636,
-0.0774915814,
-0.1903930455,
0.1450763792,
-0.0375638828,
0.0079173651,
-0.0910730362,
0.1520518214,
-0.0975839868,
-0.4702498615,
-0.2045714557,
0.1811123341,
0.0074800067,
0.0469533242,
0.4813601077,
-0.1431986392,
-0.1245555803,
-0.0785720423,
-0.2953169942,
0.0040264316,
-0.2730659246,
0.1366041899,
0.1816231608,
0.103859432,
0.0084615927,
-0.1486845016,
0.2930162251,
-0.0715196803,
0.0246398468,
0.1069570333,
0.0452330634,
-0.2920732796,
-0.2664697468,
-0.4750192463,
-0.4745301306,
-0.1774818748,
0.0669479594,
-0.075908266,
-0.0335285738,
0.5198025703,
0.0684420913,
0.0656812266,
0.0931449234,
0.023252707,
-0.3467434645,
-0.0935407355,
0.2490479201,
-0.179091692,
-0.210245356,
0.1960575432,
0.1093095243,
0.1175282896,
-0.20393309,
-0.3139223158,
0.0728625953,
-0.0576012135,
0.058944717,
0.0813103691,
0.1948962212,
0.2138828039,
0.2671021223,
-0.1480035037,
-0.3270511925,
-0.3317118883,
0.1381734908,
-0.0719676912,
0.1306350976,
-0.1062558591,
0.294085592,
-0.152083829,
0.326508671,
0.0217723008,
-0.2126558274,
0.191888988,
-0.0478476658,
0.5207321048,
0.068380706,
-0.4193751216,
0.0658852011,
-0.1191071793,
0.0269460715,
0.1760428697,
-0.3196389079,
0.0379566401,
-0.1479681134,
0.3946042061,
-0.2478049397,
-0.0996567011,
-0.1006427184,
-0.1501683444,
0.0405269749,
0.1169003174,
-0.0409751162,
-0.2084082365,
-0.1915850341,
-0.2512801886,
0.0578238554,
-0.0954614133,
-0.087551482,
-0.533575058,
-0.2334993929,
-0.1649958491,
0.2940982878,
0.1574513167,
0.3346639276,
-0.1785171777,
0.2454715222,
0.0477584898,
0.2458998859,
0.6149811745,
-0.4832390547,
0.154702425,
0.2263719141,
0.3931272328,
-0.1780015528,
-0.1272553653,
-0.1692574769,
0.2947262228,
-0.2957772911,
0.0259887744,
-0.1555344164,
-0.0910770446,
0.2631006241,
0.1554057747,
-0.1223803461,
-0.3149805069,
-0.2309516966,
-0.1793929189,
-0.1272854507,
-0.0533969514,
0.0635773465,
0.1866380721,
-0.3784870207,
0.0986478776,
0.1408236176,
0.0735073909,
0.2715045512,
0.1186348274,
0.2232429981,
0.0302767064,
0.197361365,
0.317402184,
0.2587257028,
0.5099822879,
0.5483981967,
-0.3618466854,
-0.5113294125,
-0.1194789112,
-0.1147691086,
0.2007077336,
0.0650010705,
-0.1422151178,
0.1660957336,
-0.0506398827,
0.185458824,
0.0961978808,
0.0223078672,
0.4922952354,
0.1605764627,
-0.4898479283,
-0.5388931632,
0.2713592649,
0.0392414108,
-0.0478406474,
0.1281552166,
0.0797321871,
-0.3407573402,
0.2059525549,
-0.1559936553,
0.6908729672,
0.0837977454,
-0.0510304905,
-0.0170310941,
-0.2261731625,
0.7132234573,
-0.1187111512,
0.0007714578,
-0.3614293039,
-0.3074660599,
-0.0622299947,
-0.0221186746,
0.0234751571,
0.4506933987,
-0.3085790277,
0.3788379431,
-0.3285133839,
0.2046867758,
0.028750021,
-0.0740614757,
0.2494399399,
-0.1323137879,
-0.0824863017,
0.2010339946,
-0.1576190293,
-0.1343754977,
-0.1674345434,
0.0419054106,
-0.2318485528,
-0.2680606246,
-0.2262603641,
0.08226078,
-0.2538248897,
0.1415783167,
-0.0856540874,
-0.0167195015,
0.2168119252,
0.2025102079,
0.4863218665,
0.0789240599,
-0.1482862979,
0.1838956922,
0.0421487242,
0.1370235533,
0.0343489051,
0.0537468866,
0.4150622785,
0.1160496846,
-0.2699809074,
0.1285305768,
-0.0795674771,
0.1759498864,
-0.0870310813,
0.0838943273,
-0.0983974338,
-0.3653748333,
0.0326472521,
-0.0770386755,
0.2287106365,
-0.2537733614,
0.1773460358,
0.0519831851,
-0.0466800705,
0.2120788842,
-0.2636915445,
-0.1465975195,
-0.0457466282,
0.1892329752,
0.0259641036,
0.1933565587,
0.2472447455,
0.0259730872,
-0.3513919413,
-0.2565112412,
0.038952101,
0.0239994247,
-0.6778352261,
0.2459824234,
0.2533547282,
0.0759458542,
-0.057189174,
0.2232685387,
-0.0158301294,
0.0710849985,
0.0610140152,
-0.5274376869,
-0.2186500877,
-0.00467043,
-0.1847544909,
0.1169297621,
0.2478654385,
0.4110050797,
0.1337576956,
0.0714501962,
-0.3641543388,
-0.020954119,
-0.172394067,
0.2980129123,
0.1525106579,
0.0199611541,
0.2820312381,
-0.1788062751,
0.218216911,
0.0007437372,
0.007750046,
-0.2498184741,
-0.1872092783,
0.1124106348,
0.1352093369,
-0.1972157359,
-0.0634114593,
-0.1643812805,
0.0578369349,
-0.0719840601,
-0.0172511172,
0.3105739653,
0.0032078198,
0.3735309839,
-0.1509219557,
0.2327846885,
-0.0463298075,
0.2182779461,
0.0127172945,
-0.114001587,
0.0742451549,
0.1729656607,
-0.1127646267,
0.0194780901,
-0.1750794947,
0.0847947747,
-0.1170051619,
-0.11792963,
0.1342932731,
-0.568980515,
0.2959564924,
0.193638131,
0.449362725,
0.2781369686,
-0.1868256927,
-0.0707662776,
0.3826093972,
0.307926774,
-0.3656698763,
-0.1683599651,
0.1630016863,
0.1655610651,
0.0003906599,
0.0756075159,
0.060725417,
-0.0310598556,
0.0448926538,
0.0080858618,
0.4554387927,
-0.4376546144,
0.2349053174,
0.5269825459,
-0.0619708523,
0.0999840051,
0.4967942238,
0.1731765717,
0.0635329187,
0.5881978869,
0.0346502028,
0.1773207486,
0.1874702275,
-0.1379599422,
-0.0754204541,
-0.172217235,
0.1809182614,
0.0969726592,
0.0188562367,
-0.0428314172,
-0.0954477713,
0.3011343777,
-0.3839455247,
0.0041103126,
-0.0057690036,
0.1111971214,
-0.2255034149,
-0.1060157418,
-0.1562631726,
-0.0987095013,
-0.1664459705,
-0.1973160356,
0.0140280882,
-0.1700504124,
0.3556354642,
-0.0742809027,
-0.1959641278,
-0.4565812349,
-0.186778456,
0.305419594,
0.065081425,
-0.2473097742,
0.0163199063,
0.2487559468,
-0.1455008835,
0.3213778436,
0.086239703,
0.6039388776,
0.1713752747,
0.0897912681,
-0.3410916328,
-0.0972951949,
-0.088580206,
0.1516636312,
0.215568006,
0.0459204242,
-0.0368339717,
0.3922656178,
0.2018781453,
-0.1707493216,
0.3085803986,
0.3357422054,
0.2227042168,
-0.170619756,
-0.0630670115,
-0.1339656562,
-0.2573547959,
-0.3266397119,
0.1013188735,
-0.2697949111,
0.1159574091,
0.4622558057,
-0.0415135659,
0.0840901211,
-0.2942293584,
0.1462800503,
0.1317104101,
0.3118901849,
0.2739442885,
-0.0476244502,
-0.3456508517,
0.1615712494,
-0.5773795247,
0.129460454,
-0.1186997294,
-0.1146923974,
-0.0334540457,
-0.1582231671,
0.1593217105,
0.020614991,
0.3829501867,
0.1888182312,
-0.0159400646,
-0.1086774766,
-0.1195141748,
-0.1931985319,
-0.0825988874,
-0.1219008714,
0.2010165602,
-0.3226899803,
-0.0618356615,
-0.1457770467,
0.038975928,
-0.3498083949,
0.0937143192,
0.1062969789,
-0.0201894138,
0.3603177369,
0.1459479332,
0.7699726224,
0.048815161,
0.1150308847,
-0.0913875476,
-0.353649199,
-0.4042430222,
0.3688688576,
0.3255911171,
0.2565357983,
-0.1063772365,
0.0097641386,
-0.1644826233,
0.0945067257,
-0.04354655,
0.3211485744,
-0.2544759512,
0.2145047933,
-0.0240108576,
0.0171401687,
0.0991312191,
-0.0556259044,
-0.0026464916,
0.3410544395,
-0.2067562938,
-0.6047096252,
0.6198091507,
-0.212931633,
-0.2395552248,
0.1537303925,
0.1545257866,
0.2213794589,
-0.2187473923,
-0.5100530386,
0.0247500949,
0.3773825169,
0.0190039705,
-0.4423446655,
-0.0037064492,
-0.1927573532,
0.051444225,
0.113560237,
0.3062537014,
0.1166288406,
-0.1932859123,
0.1991046965,
-0.2543466091
]
|
https://github.com/huggingface/datasets/issues/1989 | Question/problem with dataset labels | I've had versions where it worked fain. For this dataset, I had all kind of parsing issues that I couldn't understand. What I ended up doing is strip all the columns that I didn't need and also make the label 0/1.
I think one line that may have caused a problem was the csv version of this:
```crawl-data/CC-MAIN-2017-47/segments/1510934806225.78/wet/CC-MAIN-20171120203833-20171120223833-00571.warc.wet.gz Rose Blakey is an aspiring journalist. She is desperate to escape the from the small Australian town in which she lives. Rejection after rejection mean she is stuck in what she sees as a dead-end waitressing job. ^M ('Rose', '', 'Blakey') journalist F 38 journalist https://www.netgalley.com/catalog/book/121872 _ is desperate to escape the from the small Australian town in which _ lives. Rejection after rejection mean _ is stuck in what _ sees as a dead-end waitressing job. She is desperate to escape the from the small Australian town in which she lives. Rejection after rejection mean she is stuck in what she sees as a dead-end waitressing job.```
The error I got in this case is this one: https://github.com/huggingface/datasets/issues/1989#issuecomment-790842771
Note, this line was part of a much larger file and until this line I guess it was working fine. | Hi, I'm using a dataset with two labels "nurse" and "not nurse". For whatever reason (that I don't understand), I get an error that I think comes from the datasets package (using csv). Everything works fine if the labels are "nurse" and "surgeon".
This is the trace I get:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: not nurse
```
Any ideas how to fix this? For now, I'll probably make them numeric. | 197 | Question/problem with dataset labels
Hi, I'm using a dataset with two labels "nurse" and "not nurse". For whatever reason (that I don't understand), I get an error that I think comes from the datasets package (using csv). Everything works fine if the labels are "nurse" and "surgeon".
This is the trace I get:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: not nurse
```
Any ideas how to fix this? For now, I'll probably make them numeric.
I've had versions where it worked fain. For this dataset, I had all kind of parsing issues that I couldn't understand. What I ended up doing is strip all the columns that I didn't need and also make the label 0/1.
I think one line that may have caused a problem was the csv version of this:
```crawl-data/CC-MAIN-2017-47/segments/1510934806225.78/wet/CC-MAIN-20171120203833-20171120223833-00571.warc.wet.gz Rose Blakey is an aspiring journalist. She is desperate to escape the from the small Australian town in which she lives. Rejection after rejection mean she is stuck in what she sees as a dead-end waitressing job. ^M ('Rose', '', 'Blakey') journalist F 38 journalist https://www.netgalley.com/catalog/book/121872 _ is desperate to escape the from the small Australian town in which _ lives. Rejection after rejection mean _ is stuck in what _ sees as a dead-end waitressing job. She is desperate to escape the from the small Australian town in which she lives. Rejection after rejection mean she is stuck in what she sees as a dead-end waitressing job.```
The error I got in this case is this one: https://github.com/huggingface/datasets/issues/1989#issuecomment-790842771
Note, this line was part of a much larger file and until this line I guess it was working fine. | [
0.1789148301,
-0.0573230609,
0.0051793531,
0.1343671232,
0.4113537371,
0.3502124548,
0.6419945955,
0.1637074798,
-0.1613179296,
0.0723498836,
0.1788543165,
0.0638753474,
-0.0607729964,
0.030525703,
-0.130230993,
-0.1193704456,
0.0890837312,
0.1734791994,
0.1816426963,
-0.04446676,
-0.2867425382,
-0.0398259945,
-0.156679973,
0.3662885129,
-0.297205627,
-0.3766838312,
0.2105430812,
-0.1205282882,
-0.1131331921,
-0.4633954763,
0.2718095183,
0.0861200094,
0.0026800728,
0.4034268558,
-0.0001030963,
0.058756806,
0.2967477739,
0.1559889019,
-0.3984076679,
-0.4321287572,
-0.1893597841,
-0.0234080795,
0.0345638432,
-0.3044767976,
0.0979833305,
-0.2846682072,
-0.0905410498,
-0.2756658196,
0.1046864912,
0.4386059344,
0.3266399205,
0.2373396754,
-0.2065001428,
-0.2293031216,
0.2311526835,
0.1250885725,
-0.0305089504,
0.0494753644,
-0.1344386041,
-0.0064582187,
0.4515704513,
0.460914433,
-0.2723956704,
0.2647709846,
0.1353648007,
0.1463635266,
0.1377247274,
-0.3910481632,
0.2763096094,
0.1961432248,
0.7452604771,
-0.2964998186,
-0.2943911254,
-0.1697053015,
0.1373766065,
-0.4167195559,
0.1813390404,
0.0700832456,
0.0063756779,
-0.0470148437,
-0.0331627578,
0.0610270314,
0.0807417855,
0.0881804153,
-0.0580748878,
0.0383284204,
-0.0986412764,
0.3288168907,
0.1125301495,
-0.0747507066,
0.2975301445,
-0.0059941988,
-0.0885701999,
0.0415449142,
-0.4325241745,
-0.0296084415,
-0.0368810184,
0.0516759381,
-0.1181376055,
-0.038740769,
0.0183869507,
-0.3749563098,
-0.1474356651,
0.3403449953,
0.2151344717,
0.2662764788,
0.2706995606,
0.5616863966,
0.0911723822,
-0.0761627182,
0.0308040548,
-0.0558074787,
-0.0015739995,
-0.4626432061,
0.2433210015,
0.0989312604,
0.2934703231,
-0.2398585975,
-0.5455213189,
0.1924421936,
-0.0281014666,
0.0940956026,
0.1541178226,
0.3049503565,
0.0221220106,
0.2065178901,
0.0793469325,
0.193608433,
-0.0091703041,
-0.1172054783,
-0.2069241703,
0.0976080745,
-0.2002791017,
-0.150106892,
0.0502697304,
-0.0720530748,
0.1124490499,
0.0640597194,
-0.0010049042,
-0.0760566816,
-0.0163034722,
-0.3864641488,
-0.012748206,
0.3588934243,
-0.1032633781,
0.420350641,
0.2520543337,
-0.4185501039,
0.0596993491,
0.1802113652,
-0.3688521683,
-0.0338135473,
-0.2121574432,
0.2862125635,
-0.1030441746,
-0.187981084,
-0.1200913265,
0.0468237363,
0.4193198979,
-0.2145542502,
0.1575898081,
-0.3543840945,
-0.3093222678,
-0.2777702212,
-0.0663038418,
0.2214321792,
-0.6722466946,
0.004389429,
-0.1475467384,
-0.0291129835,
0.0528065376,
0.2960194051,
-0.0810041502,
0.2062916607,
-0.0705919117,
0.0303497873,
0.1395514011,
-0.390691489,
-0.1104637682,
0.1035439447,
-0.1420033723,
-0.2442642748,
0.0704807937,
-0.0403395221,
0.0917313397,
-0.0916260481,
0.0582318865,
-0.1318774968,
-0.1996321678,
-0.0951335654,
-0.1437036842,
0.0538111962,
0.653909564,
0.0927327722,
-0.016800072,
0.0756470934,
-0.1002285406,
-0.3448368311,
-0.0425327159,
-0.1009554341,
0.2062197328,
0.2147901356,
0.0556404777,
0.2983597219,
0.1459521502,
-0.0644702911,
-0.4424965978,
0.1017011777,
-0.0400518253,
0.1395645589,
-0.145143196,
-0.1923438162,
-0.3297647238,
-0.0537859611,
-0.2079762369,
0.0183238313,
0.1633132249,
0.0683607757,
-0.2214221507,
-0.0204832722,
0.0140843056,
-0.075884521,
-0.0172140431,
0.1093305945,
-0.1040251106,
0.2656517625,
-0.0951162279,
0.1168940216,
-0.0267393459,
0.2531200051,
0.4326063693,
0.2400517166,
-0.0466214195,
0.367023617,
0.0848606005,
-0.3270231187,
0.0078404322,
-0.172658667,
0.0181292761,
-0.0838742778,
-0.0163266249,
0.1145247817,
0.2768396139,
-0.1085973084,
-0.0847191364,
0.1411861628,
-0.1772004515,
0.2762841582,
-0.1619747579,
0.2004606873,
0.2599629462,
-0.1093928516,
0.1251222938,
-0.2127112299,
0.2158810794,
-0.1297985166,
0.2343143374,
0.007142223,
-0.2617177367,
0.0677190349,
0.4220539927,
0.0015310015,
-0.0035239446,
0.009774005,
-0.1586676836,
0.1657464355,
-0.0130180242,
0.595718503,
0.352842629,
0.2059556991,
-0.1638759673,
-0.0582683198,
-0.2622588873,
-0.1369839609,
0.2124615908,
0.0951014385,
0.0298523773,
0.0572962686,
0.0261101257,
-0.0664390028,
-0.1765725613,
-0.2433390021,
0.2082311809,
0.3464297354,
-0.51031214,
-0.0029065625,
-0.3452727795,
-0.2318637222,
-0.3185971677,
-0.0987910777,
-0.2351591289,
-0.4590440094,
0.0904379636,
-0.0774915814,
-0.1903930455,
0.1450763792,
-0.0375638828,
0.0079173651,
-0.0910730362,
0.1520518214,
-0.0975839868,
-0.4702498615,
-0.2045714557,
0.1811123341,
0.0074800067,
0.0469533242,
0.4813601077,
-0.1431986392,
-0.1245555803,
-0.0785720423,
-0.2953169942,
0.0040264316,
-0.2730659246,
0.1366041899,
0.1816231608,
0.103859432,
0.0084615927,
-0.1486845016,
0.2930162251,
-0.0715196803,
0.0246398468,
0.1069570333,
0.0452330634,
-0.2920732796,
-0.2664697468,
-0.4750192463,
-0.4745301306,
-0.1774818748,
0.0669479594,
-0.075908266,
-0.0335285738,
0.5198025703,
0.0684420913,
0.0656812266,
0.0931449234,
0.023252707,
-0.3467434645,
-0.0935407355,
0.2490479201,
-0.179091692,
-0.210245356,
0.1960575432,
0.1093095243,
0.1175282896,
-0.20393309,
-0.3139223158,
0.0728625953,
-0.0576012135,
0.058944717,
0.0813103691,
0.1948962212,
0.2138828039,
0.2671021223,
-0.1480035037,
-0.3270511925,
-0.3317118883,
0.1381734908,
-0.0719676912,
0.1306350976,
-0.1062558591,
0.294085592,
-0.152083829,
0.326508671,
0.0217723008,
-0.2126558274,
0.191888988,
-0.0478476658,
0.5207321048,
0.068380706,
-0.4193751216,
0.0658852011,
-0.1191071793,
0.0269460715,
0.1760428697,
-0.3196389079,
0.0379566401,
-0.1479681134,
0.3946042061,
-0.2478049397,
-0.0996567011,
-0.1006427184,
-0.1501683444,
0.0405269749,
0.1169003174,
-0.0409751162,
-0.2084082365,
-0.1915850341,
-0.2512801886,
0.0578238554,
-0.0954614133,
-0.087551482,
-0.533575058,
-0.2334993929,
-0.1649958491,
0.2940982878,
0.1574513167,
0.3346639276,
-0.1785171777,
0.2454715222,
0.0477584898,
0.2458998859,
0.6149811745,
-0.4832390547,
0.154702425,
0.2263719141,
0.3931272328,
-0.1780015528,
-0.1272553653,
-0.1692574769,
0.2947262228,
-0.2957772911,
0.0259887744,
-0.1555344164,
-0.0910770446,
0.2631006241,
0.1554057747,
-0.1223803461,
-0.3149805069,
-0.2309516966,
-0.1793929189,
-0.1272854507,
-0.0533969514,
0.0635773465,
0.1866380721,
-0.3784870207,
0.0986478776,
0.1408236176,
0.0735073909,
0.2715045512,
0.1186348274,
0.2232429981,
0.0302767064,
0.197361365,
0.317402184,
0.2587257028,
0.5099822879,
0.5483981967,
-0.3618466854,
-0.5113294125,
-0.1194789112,
-0.1147691086,
0.2007077336,
0.0650010705,
-0.1422151178,
0.1660957336,
-0.0506398827,
0.185458824,
0.0961978808,
0.0223078672,
0.4922952354,
0.1605764627,
-0.4898479283,
-0.5388931632,
0.2713592649,
0.0392414108,
-0.0478406474,
0.1281552166,
0.0797321871,
-0.3407573402,
0.2059525549,
-0.1559936553,
0.6908729672,
0.0837977454,
-0.0510304905,
-0.0170310941,
-0.2261731625,
0.7132234573,
-0.1187111512,
0.0007714578,
-0.3614293039,
-0.3074660599,
-0.0622299947,
-0.0221186746,
0.0234751571,
0.4506933987,
-0.3085790277,
0.3788379431,
-0.3285133839,
0.2046867758,
0.028750021,
-0.0740614757,
0.2494399399,
-0.1323137879,
-0.0824863017,
0.2010339946,
-0.1576190293,
-0.1343754977,
-0.1674345434,
0.0419054106,
-0.2318485528,
-0.2680606246,
-0.2262603641,
0.08226078,
-0.2538248897,
0.1415783167,
-0.0856540874,
-0.0167195015,
0.2168119252,
0.2025102079,
0.4863218665,
0.0789240599,
-0.1482862979,
0.1838956922,
0.0421487242,
0.1370235533,
0.0343489051,
0.0537468866,
0.4150622785,
0.1160496846,
-0.2699809074,
0.1285305768,
-0.0795674771,
0.1759498864,
-0.0870310813,
0.0838943273,
-0.0983974338,
-0.3653748333,
0.0326472521,
-0.0770386755,
0.2287106365,
-0.2537733614,
0.1773460358,
0.0519831851,
-0.0466800705,
0.2120788842,
-0.2636915445,
-0.1465975195,
-0.0457466282,
0.1892329752,
0.0259641036,
0.1933565587,
0.2472447455,
0.0259730872,
-0.3513919413,
-0.2565112412,
0.038952101,
0.0239994247,
-0.6778352261,
0.2459824234,
0.2533547282,
0.0759458542,
-0.057189174,
0.2232685387,
-0.0158301294,
0.0710849985,
0.0610140152,
-0.5274376869,
-0.2186500877,
-0.00467043,
-0.1847544909,
0.1169297621,
0.2478654385,
0.4110050797,
0.1337576956,
0.0714501962,
-0.3641543388,
-0.020954119,
-0.172394067,
0.2980129123,
0.1525106579,
0.0199611541,
0.2820312381,
-0.1788062751,
0.218216911,
0.0007437372,
0.007750046,
-0.2498184741,
-0.1872092783,
0.1124106348,
0.1352093369,
-0.1972157359,
-0.0634114593,
-0.1643812805,
0.0578369349,
-0.0719840601,
-0.0172511172,
0.3105739653,
0.0032078198,
0.3735309839,
-0.1509219557,
0.2327846885,
-0.0463298075,
0.2182779461,
0.0127172945,
-0.114001587,
0.0742451549,
0.1729656607,
-0.1127646267,
0.0194780901,
-0.1750794947,
0.0847947747,
-0.1170051619,
-0.11792963,
0.1342932731,
-0.568980515,
0.2959564924,
0.193638131,
0.449362725,
0.2781369686,
-0.1868256927,
-0.0707662776,
0.3826093972,
0.307926774,
-0.3656698763,
-0.1683599651,
0.1630016863,
0.1655610651,
0.0003906599,
0.0756075159,
0.060725417,
-0.0310598556,
0.0448926538,
0.0080858618,
0.4554387927,
-0.4376546144,
0.2349053174,
0.5269825459,
-0.0619708523,
0.0999840051,
0.4967942238,
0.1731765717,
0.0635329187,
0.5881978869,
0.0346502028,
0.1773207486,
0.1874702275,
-0.1379599422,
-0.0754204541,
-0.172217235,
0.1809182614,
0.0969726592,
0.0188562367,
-0.0428314172,
-0.0954477713,
0.3011343777,
-0.3839455247,
0.0041103126,
-0.0057690036,
0.1111971214,
-0.2255034149,
-0.1060157418,
-0.1562631726,
-0.0987095013,
-0.1664459705,
-0.1973160356,
0.0140280882,
-0.1700504124,
0.3556354642,
-0.0742809027,
-0.1959641278,
-0.4565812349,
-0.186778456,
0.305419594,
0.065081425,
-0.2473097742,
0.0163199063,
0.2487559468,
-0.1455008835,
0.3213778436,
0.086239703,
0.6039388776,
0.1713752747,
0.0897912681,
-0.3410916328,
-0.0972951949,
-0.088580206,
0.1516636312,
0.215568006,
0.0459204242,
-0.0368339717,
0.3922656178,
0.2018781453,
-0.1707493216,
0.3085803986,
0.3357422054,
0.2227042168,
-0.170619756,
-0.0630670115,
-0.1339656562,
-0.2573547959,
-0.3266397119,
0.1013188735,
-0.2697949111,
0.1159574091,
0.4622558057,
-0.0415135659,
0.0840901211,
-0.2942293584,
0.1462800503,
0.1317104101,
0.3118901849,
0.2739442885,
-0.0476244502,
-0.3456508517,
0.1615712494,
-0.5773795247,
0.129460454,
-0.1186997294,
-0.1146923974,
-0.0334540457,
-0.1582231671,
0.1593217105,
0.020614991,
0.3829501867,
0.1888182312,
-0.0159400646,
-0.1086774766,
-0.1195141748,
-0.1931985319,
-0.0825988874,
-0.1219008714,
0.2010165602,
-0.3226899803,
-0.0618356615,
-0.1457770467,
0.038975928,
-0.3498083949,
0.0937143192,
0.1062969789,
-0.0201894138,
0.3603177369,
0.1459479332,
0.7699726224,
0.048815161,
0.1150308847,
-0.0913875476,
-0.353649199,
-0.4042430222,
0.3688688576,
0.3255911171,
0.2565357983,
-0.1063772365,
0.0097641386,
-0.1644826233,
0.0945067257,
-0.04354655,
0.3211485744,
-0.2544759512,
0.2145047933,
-0.0240108576,
0.0171401687,
0.0991312191,
-0.0556259044,
-0.0026464916,
0.3410544395,
-0.2067562938,
-0.6047096252,
0.6198091507,
-0.212931633,
-0.2395552248,
0.1537303925,
0.1545257866,
0.2213794589,
-0.2187473923,
-0.5100530386,
0.0247500949,
0.3773825169,
0.0190039705,
-0.4423446655,
-0.0037064492,
-0.1927573532,
0.051444225,
0.113560237,
0.3062537014,
0.1166288406,
-0.1932859123,
0.1991046965,
-0.2543466091
]
|
https://github.com/huggingface/datasets/issues/1989 | Question/problem with dataset labels | Hi @ioana-blue,
What is the separator you're using for the csv? I see there are only two commas in the given line, but they don't seem like appropriate points. Also, is this a string part of one line, or an entire line? There should also be a label, right? | Hi, I'm using a dataset with two labels "nurse" and "not nurse". For whatever reason (that I don't understand), I get an error that I think comes from the datasets package (using csv). Everything works fine if the labels are "nurse" and "surgeon".
This is the trace I get:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: not nurse
```
Any ideas how to fix this? For now, I'll probably make them numeric. | 49 | Question/problem with dataset labels
Hi, I'm using a dataset with two labels "nurse" and "not nurse". For whatever reason (that I don't understand), I get an error that I think comes from the datasets package (using csv). Everything works fine if the labels are "nurse" and "surgeon".
This is the trace I get:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: not nurse
```
Any ideas how to fix this? For now, I'll probably make them numeric.
Hi @ioana-blue,
What is the separator you're using for the csv? I see there are only two commas in the given line, but they don't seem like appropriate points. Also, is this a string part of one line, or an entire line? There should also be a label, right? | [
0.1789148301,
-0.0573230609,
0.0051793531,
0.1343671232,
0.4113537371,
0.3502124548,
0.6419945955,
0.1637074798,
-0.1613179296,
0.0723498836,
0.1788543165,
0.0638753474,
-0.0607729964,
0.030525703,
-0.130230993,
-0.1193704456,
0.0890837312,
0.1734791994,
0.1816426963,
-0.04446676,
-0.2867425382,
-0.0398259945,
-0.156679973,
0.3662885129,
-0.297205627,
-0.3766838312,
0.2105430812,
-0.1205282882,
-0.1131331921,
-0.4633954763,
0.2718095183,
0.0861200094,
0.0026800728,
0.4034268558,
-0.0001030963,
0.058756806,
0.2967477739,
0.1559889019,
-0.3984076679,
-0.4321287572,
-0.1893597841,
-0.0234080795,
0.0345638432,
-0.3044767976,
0.0979833305,
-0.2846682072,
-0.0905410498,
-0.2756658196,
0.1046864912,
0.4386059344,
0.3266399205,
0.2373396754,
-0.2065001428,
-0.2293031216,
0.2311526835,
0.1250885725,
-0.0305089504,
0.0494753644,
-0.1344386041,
-0.0064582187,
0.4515704513,
0.460914433,
-0.2723956704,
0.2647709846,
0.1353648007,
0.1463635266,
0.1377247274,
-0.3910481632,
0.2763096094,
0.1961432248,
0.7452604771,
-0.2964998186,
-0.2943911254,
-0.1697053015,
0.1373766065,
-0.4167195559,
0.1813390404,
0.0700832456,
0.0063756779,
-0.0470148437,
-0.0331627578,
0.0610270314,
0.0807417855,
0.0881804153,
-0.0580748878,
0.0383284204,
-0.0986412764,
0.3288168907,
0.1125301495,
-0.0747507066,
0.2975301445,
-0.0059941988,
-0.0885701999,
0.0415449142,
-0.4325241745,
-0.0296084415,
-0.0368810184,
0.0516759381,
-0.1181376055,
-0.038740769,
0.0183869507,
-0.3749563098,
-0.1474356651,
0.3403449953,
0.2151344717,
0.2662764788,
0.2706995606,
0.5616863966,
0.0911723822,
-0.0761627182,
0.0308040548,
-0.0558074787,
-0.0015739995,
-0.4626432061,
0.2433210015,
0.0989312604,
0.2934703231,
-0.2398585975,
-0.5455213189,
0.1924421936,
-0.0281014666,
0.0940956026,
0.1541178226,
0.3049503565,
0.0221220106,
0.2065178901,
0.0793469325,
0.193608433,
-0.0091703041,
-0.1172054783,
-0.2069241703,
0.0976080745,
-0.2002791017,
-0.150106892,
0.0502697304,
-0.0720530748,
0.1124490499,
0.0640597194,
-0.0010049042,
-0.0760566816,
-0.0163034722,
-0.3864641488,
-0.012748206,
0.3588934243,
-0.1032633781,
0.420350641,
0.2520543337,
-0.4185501039,
0.0596993491,
0.1802113652,
-0.3688521683,
-0.0338135473,
-0.2121574432,
0.2862125635,
-0.1030441746,
-0.187981084,
-0.1200913265,
0.0468237363,
0.4193198979,
-0.2145542502,
0.1575898081,
-0.3543840945,
-0.3093222678,
-0.2777702212,
-0.0663038418,
0.2214321792,
-0.6722466946,
0.004389429,
-0.1475467384,
-0.0291129835,
0.0528065376,
0.2960194051,
-0.0810041502,
0.2062916607,
-0.0705919117,
0.0303497873,
0.1395514011,
-0.390691489,
-0.1104637682,
0.1035439447,
-0.1420033723,
-0.2442642748,
0.0704807937,
-0.0403395221,
0.0917313397,
-0.0916260481,
0.0582318865,
-0.1318774968,
-0.1996321678,
-0.0951335654,
-0.1437036842,
0.0538111962,
0.653909564,
0.0927327722,
-0.016800072,
0.0756470934,
-0.1002285406,
-0.3448368311,
-0.0425327159,
-0.1009554341,
0.2062197328,
0.2147901356,
0.0556404777,
0.2983597219,
0.1459521502,
-0.0644702911,
-0.4424965978,
0.1017011777,
-0.0400518253,
0.1395645589,
-0.145143196,
-0.1923438162,
-0.3297647238,
-0.0537859611,
-0.2079762369,
0.0183238313,
0.1633132249,
0.0683607757,
-0.2214221507,
-0.0204832722,
0.0140843056,
-0.075884521,
-0.0172140431,
0.1093305945,
-0.1040251106,
0.2656517625,
-0.0951162279,
0.1168940216,
-0.0267393459,
0.2531200051,
0.4326063693,
0.2400517166,
-0.0466214195,
0.367023617,
0.0848606005,
-0.3270231187,
0.0078404322,
-0.172658667,
0.0181292761,
-0.0838742778,
-0.0163266249,
0.1145247817,
0.2768396139,
-0.1085973084,
-0.0847191364,
0.1411861628,
-0.1772004515,
0.2762841582,
-0.1619747579,
0.2004606873,
0.2599629462,
-0.1093928516,
0.1251222938,
-0.2127112299,
0.2158810794,
-0.1297985166,
0.2343143374,
0.007142223,
-0.2617177367,
0.0677190349,
0.4220539927,
0.0015310015,
-0.0035239446,
0.009774005,
-0.1586676836,
0.1657464355,
-0.0130180242,
0.595718503,
0.352842629,
0.2059556991,
-0.1638759673,
-0.0582683198,
-0.2622588873,
-0.1369839609,
0.2124615908,
0.0951014385,
0.0298523773,
0.0572962686,
0.0261101257,
-0.0664390028,
-0.1765725613,
-0.2433390021,
0.2082311809,
0.3464297354,
-0.51031214,
-0.0029065625,
-0.3452727795,
-0.2318637222,
-0.3185971677,
-0.0987910777,
-0.2351591289,
-0.4590440094,
0.0904379636,
-0.0774915814,
-0.1903930455,
0.1450763792,
-0.0375638828,
0.0079173651,
-0.0910730362,
0.1520518214,
-0.0975839868,
-0.4702498615,
-0.2045714557,
0.1811123341,
0.0074800067,
0.0469533242,
0.4813601077,
-0.1431986392,
-0.1245555803,
-0.0785720423,
-0.2953169942,
0.0040264316,
-0.2730659246,
0.1366041899,
0.1816231608,
0.103859432,
0.0084615927,
-0.1486845016,
0.2930162251,
-0.0715196803,
0.0246398468,
0.1069570333,
0.0452330634,
-0.2920732796,
-0.2664697468,
-0.4750192463,
-0.4745301306,
-0.1774818748,
0.0669479594,
-0.075908266,
-0.0335285738,
0.5198025703,
0.0684420913,
0.0656812266,
0.0931449234,
0.023252707,
-0.3467434645,
-0.0935407355,
0.2490479201,
-0.179091692,
-0.210245356,
0.1960575432,
0.1093095243,
0.1175282896,
-0.20393309,
-0.3139223158,
0.0728625953,
-0.0576012135,
0.058944717,
0.0813103691,
0.1948962212,
0.2138828039,
0.2671021223,
-0.1480035037,
-0.3270511925,
-0.3317118883,
0.1381734908,
-0.0719676912,
0.1306350976,
-0.1062558591,
0.294085592,
-0.152083829,
0.326508671,
0.0217723008,
-0.2126558274,
0.191888988,
-0.0478476658,
0.5207321048,
0.068380706,
-0.4193751216,
0.0658852011,
-0.1191071793,
0.0269460715,
0.1760428697,
-0.3196389079,
0.0379566401,
-0.1479681134,
0.3946042061,
-0.2478049397,
-0.0996567011,
-0.1006427184,
-0.1501683444,
0.0405269749,
0.1169003174,
-0.0409751162,
-0.2084082365,
-0.1915850341,
-0.2512801886,
0.0578238554,
-0.0954614133,
-0.087551482,
-0.533575058,
-0.2334993929,
-0.1649958491,
0.2940982878,
0.1574513167,
0.3346639276,
-0.1785171777,
0.2454715222,
0.0477584898,
0.2458998859,
0.6149811745,
-0.4832390547,
0.154702425,
0.2263719141,
0.3931272328,
-0.1780015528,
-0.1272553653,
-0.1692574769,
0.2947262228,
-0.2957772911,
0.0259887744,
-0.1555344164,
-0.0910770446,
0.2631006241,
0.1554057747,
-0.1223803461,
-0.3149805069,
-0.2309516966,
-0.1793929189,
-0.1272854507,
-0.0533969514,
0.0635773465,
0.1866380721,
-0.3784870207,
0.0986478776,
0.1408236176,
0.0735073909,
0.2715045512,
0.1186348274,
0.2232429981,
0.0302767064,
0.197361365,
0.317402184,
0.2587257028,
0.5099822879,
0.5483981967,
-0.3618466854,
-0.5113294125,
-0.1194789112,
-0.1147691086,
0.2007077336,
0.0650010705,
-0.1422151178,
0.1660957336,
-0.0506398827,
0.185458824,
0.0961978808,
0.0223078672,
0.4922952354,
0.1605764627,
-0.4898479283,
-0.5388931632,
0.2713592649,
0.0392414108,
-0.0478406474,
0.1281552166,
0.0797321871,
-0.3407573402,
0.2059525549,
-0.1559936553,
0.6908729672,
0.0837977454,
-0.0510304905,
-0.0170310941,
-0.2261731625,
0.7132234573,
-0.1187111512,
0.0007714578,
-0.3614293039,
-0.3074660599,
-0.0622299947,
-0.0221186746,
0.0234751571,
0.4506933987,
-0.3085790277,
0.3788379431,
-0.3285133839,
0.2046867758,
0.028750021,
-0.0740614757,
0.2494399399,
-0.1323137879,
-0.0824863017,
0.2010339946,
-0.1576190293,
-0.1343754977,
-0.1674345434,
0.0419054106,
-0.2318485528,
-0.2680606246,
-0.2262603641,
0.08226078,
-0.2538248897,
0.1415783167,
-0.0856540874,
-0.0167195015,
0.2168119252,
0.2025102079,
0.4863218665,
0.0789240599,
-0.1482862979,
0.1838956922,
0.0421487242,
0.1370235533,
0.0343489051,
0.0537468866,
0.4150622785,
0.1160496846,
-0.2699809074,
0.1285305768,
-0.0795674771,
0.1759498864,
-0.0870310813,
0.0838943273,
-0.0983974338,
-0.3653748333,
0.0326472521,
-0.0770386755,
0.2287106365,
-0.2537733614,
0.1773460358,
0.0519831851,
-0.0466800705,
0.2120788842,
-0.2636915445,
-0.1465975195,
-0.0457466282,
0.1892329752,
0.0259641036,
0.1933565587,
0.2472447455,
0.0259730872,
-0.3513919413,
-0.2565112412,
0.038952101,
0.0239994247,
-0.6778352261,
0.2459824234,
0.2533547282,
0.0759458542,
-0.057189174,
0.2232685387,
-0.0158301294,
0.0710849985,
0.0610140152,
-0.5274376869,
-0.2186500877,
-0.00467043,
-0.1847544909,
0.1169297621,
0.2478654385,
0.4110050797,
0.1337576956,
0.0714501962,
-0.3641543388,
-0.020954119,
-0.172394067,
0.2980129123,
0.1525106579,
0.0199611541,
0.2820312381,
-0.1788062751,
0.218216911,
0.0007437372,
0.007750046,
-0.2498184741,
-0.1872092783,
0.1124106348,
0.1352093369,
-0.1972157359,
-0.0634114593,
-0.1643812805,
0.0578369349,
-0.0719840601,
-0.0172511172,
0.3105739653,
0.0032078198,
0.3735309839,
-0.1509219557,
0.2327846885,
-0.0463298075,
0.2182779461,
0.0127172945,
-0.114001587,
0.0742451549,
0.1729656607,
-0.1127646267,
0.0194780901,
-0.1750794947,
0.0847947747,
-0.1170051619,
-0.11792963,
0.1342932731,
-0.568980515,
0.2959564924,
0.193638131,
0.449362725,
0.2781369686,
-0.1868256927,
-0.0707662776,
0.3826093972,
0.307926774,
-0.3656698763,
-0.1683599651,
0.1630016863,
0.1655610651,
0.0003906599,
0.0756075159,
0.060725417,
-0.0310598556,
0.0448926538,
0.0080858618,
0.4554387927,
-0.4376546144,
0.2349053174,
0.5269825459,
-0.0619708523,
0.0999840051,
0.4967942238,
0.1731765717,
0.0635329187,
0.5881978869,
0.0346502028,
0.1773207486,
0.1874702275,
-0.1379599422,
-0.0754204541,
-0.172217235,
0.1809182614,
0.0969726592,
0.0188562367,
-0.0428314172,
-0.0954477713,
0.3011343777,
-0.3839455247,
0.0041103126,
-0.0057690036,
0.1111971214,
-0.2255034149,
-0.1060157418,
-0.1562631726,
-0.0987095013,
-0.1664459705,
-0.1973160356,
0.0140280882,
-0.1700504124,
0.3556354642,
-0.0742809027,
-0.1959641278,
-0.4565812349,
-0.186778456,
0.305419594,
0.065081425,
-0.2473097742,
0.0163199063,
0.2487559468,
-0.1455008835,
0.3213778436,
0.086239703,
0.6039388776,
0.1713752747,
0.0897912681,
-0.3410916328,
-0.0972951949,
-0.088580206,
0.1516636312,
0.215568006,
0.0459204242,
-0.0368339717,
0.3922656178,
0.2018781453,
-0.1707493216,
0.3085803986,
0.3357422054,
0.2227042168,
-0.170619756,
-0.0630670115,
-0.1339656562,
-0.2573547959,
-0.3266397119,
0.1013188735,
-0.2697949111,
0.1159574091,
0.4622558057,
-0.0415135659,
0.0840901211,
-0.2942293584,
0.1462800503,
0.1317104101,
0.3118901849,
0.2739442885,
-0.0476244502,
-0.3456508517,
0.1615712494,
-0.5773795247,
0.129460454,
-0.1186997294,
-0.1146923974,
-0.0334540457,
-0.1582231671,
0.1593217105,
0.020614991,
0.3829501867,
0.1888182312,
-0.0159400646,
-0.1086774766,
-0.1195141748,
-0.1931985319,
-0.0825988874,
-0.1219008714,
0.2010165602,
-0.3226899803,
-0.0618356615,
-0.1457770467,
0.038975928,
-0.3498083949,
0.0937143192,
0.1062969789,
-0.0201894138,
0.3603177369,
0.1459479332,
0.7699726224,
0.048815161,
0.1150308847,
-0.0913875476,
-0.353649199,
-0.4042430222,
0.3688688576,
0.3255911171,
0.2565357983,
-0.1063772365,
0.0097641386,
-0.1644826233,
0.0945067257,
-0.04354655,
0.3211485744,
-0.2544759512,
0.2145047933,
-0.0240108576,
0.0171401687,
0.0991312191,
-0.0556259044,
-0.0026464916,
0.3410544395,
-0.2067562938,
-0.6047096252,
0.6198091507,
-0.212931633,
-0.2395552248,
0.1537303925,
0.1545257866,
0.2213794589,
-0.2187473923,
-0.5100530386,
0.0247500949,
0.3773825169,
0.0190039705,
-0.4423446655,
-0.0037064492,
-0.1927573532,
0.051444225,
0.113560237,
0.3062537014,
0.1166288406,
-0.1932859123,
0.1991046965,
-0.2543466091
]
|
https://github.com/huggingface/datasets/issues/1989 | Question/problem with dataset labels | Sorry for the confusion, the sample above was from a tsv that was used to derive the csv. Let me construct the csv again (I had remove it).
This is the line in the csv - this is the whole line:
```crawl-data/CC-MAIN-2017-47/segments/1510934806225.78/wet/CC-MAIN-20171120203833-20171120223833-00571.warc.wet.gz,Rose Blakey is an aspiring journalist. She is desperate to escape the from the small Australian town in which she lives. Rejection after rejection mean she is stuck in what she sees as a dead,"('Rose', '', 'Blakey')",journalist,F,38,journalist,https://www.netgalley.com/catalog/book/121872,_ is desperate to escape the from the small Australian town in which _ lives. Rejection after rejection mean _ is stuck in what _ sees as a dead-end waitressing job., She is desperate to escape the from the small Australian town in which she lives. Rejection after rejection mean she is stuck in what she sees as a dead-end waitressing job.``` | Hi, I'm using a dataset with two labels "nurse" and "not nurse". For whatever reason (that I don't understand), I get an error that I think comes from the datasets package (using csv). Everything works fine if the labels are "nurse" and "surgeon".
This is the trace I get:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: not nurse
```
Any ideas how to fix this? For now, I'll probably make them numeric. | 139 | Question/problem with dataset labels
Hi, I'm using a dataset with two labels "nurse" and "not nurse". For whatever reason (that I don't understand), I get an error that I think comes from the datasets package (using csv). Everything works fine if the labels are "nurse" and "surgeon".
This is the trace I get:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: not nurse
```
Any ideas how to fix this? For now, I'll probably make them numeric.
Sorry for the confusion, the sample above was from a tsv that was used to derive the csv. Let me construct the csv again (I had remove it).
This is the line in the csv - this is the whole line:
```crawl-data/CC-MAIN-2017-47/segments/1510934806225.78/wet/CC-MAIN-20171120203833-20171120223833-00571.warc.wet.gz,Rose Blakey is an aspiring journalist. She is desperate to escape the from the small Australian town in which she lives. Rejection after rejection mean she is stuck in what she sees as a dead,"('Rose', '', 'Blakey')",journalist,F,38,journalist,https://www.netgalley.com/catalog/book/121872,_ is desperate to escape the from the small Australian town in which _ lives. Rejection after rejection mean _ is stuck in what _ sees as a dead-end waitressing job., She is desperate to escape the from the small Australian town in which she lives. Rejection after rejection mean she is stuck in what she sees as a dead-end waitressing job.``` | [
0.1789148301,
-0.0573230609,
0.0051793531,
0.1343671232,
0.4113537371,
0.3502124548,
0.6419945955,
0.1637074798,
-0.1613179296,
0.0723498836,
0.1788543165,
0.0638753474,
-0.0607729964,
0.030525703,
-0.130230993,
-0.1193704456,
0.0890837312,
0.1734791994,
0.1816426963,
-0.04446676,
-0.2867425382,
-0.0398259945,
-0.156679973,
0.3662885129,
-0.297205627,
-0.3766838312,
0.2105430812,
-0.1205282882,
-0.1131331921,
-0.4633954763,
0.2718095183,
0.0861200094,
0.0026800728,
0.4034268558,
-0.0001030963,
0.058756806,
0.2967477739,
0.1559889019,
-0.3984076679,
-0.4321287572,
-0.1893597841,
-0.0234080795,
0.0345638432,
-0.3044767976,
0.0979833305,
-0.2846682072,
-0.0905410498,
-0.2756658196,
0.1046864912,
0.4386059344,
0.3266399205,
0.2373396754,
-0.2065001428,
-0.2293031216,
0.2311526835,
0.1250885725,
-0.0305089504,
0.0494753644,
-0.1344386041,
-0.0064582187,
0.4515704513,
0.460914433,
-0.2723956704,
0.2647709846,
0.1353648007,
0.1463635266,
0.1377247274,
-0.3910481632,
0.2763096094,
0.1961432248,
0.7452604771,
-0.2964998186,
-0.2943911254,
-0.1697053015,
0.1373766065,
-0.4167195559,
0.1813390404,
0.0700832456,
0.0063756779,
-0.0470148437,
-0.0331627578,
0.0610270314,
0.0807417855,
0.0881804153,
-0.0580748878,
0.0383284204,
-0.0986412764,
0.3288168907,
0.1125301495,
-0.0747507066,
0.2975301445,
-0.0059941988,
-0.0885701999,
0.0415449142,
-0.4325241745,
-0.0296084415,
-0.0368810184,
0.0516759381,
-0.1181376055,
-0.038740769,
0.0183869507,
-0.3749563098,
-0.1474356651,
0.3403449953,
0.2151344717,
0.2662764788,
0.2706995606,
0.5616863966,
0.0911723822,
-0.0761627182,
0.0308040548,
-0.0558074787,
-0.0015739995,
-0.4626432061,
0.2433210015,
0.0989312604,
0.2934703231,
-0.2398585975,
-0.5455213189,
0.1924421936,
-0.0281014666,
0.0940956026,
0.1541178226,
0.3049503565,
0.0221220106,
0.2065178901,
0.0793469325,
0.193608433,
-0.0091703041,
-0.1172054783,
-0.2069241703,
0.0976080745,
-0.2002791017,
-0.150106892,
0.0502697304,
-0.0720530748,
0.1124490499,
0.0640597194,
-0.0010049042,
-0.0760566816,
-0.0163034722,
-0.3864641488,
-0.012748206,
0.3588934243,
-0.1032633781,
0.420350641,
0.2520543337,
-0.4185501039,
0.0596993491,
0.1802113652,
-0.3688521683,
-0.0338135473,
-0.2121574432,
0.2862125635,
-0.1030441746,
-0.187981084,
-0.1200913265,
0.0468237363,
0.4193198979,
-0.2145542502,
0.1575898081,
-0.3543840945,
-0.3093222678,
-0.2777702212,
-0.0663038418,
0.2214321792,
-0.6722466946,
0.004389429,
-0.1475467384,
-0.0291129835,
0.0528065376,
0.2960194051,
-0.0810041502,
0.2062916607,
-0.0705919117,
0.0303497873,
0.1395514011,
-0.390691489,
-0.1104637682,
0.1035439447,
-0.1420033723,
-0.2442642748,
0.0704807937,
-0.0403395221,
0.0917313397,
-0.0916260481,
0.0582318865,
-0.1318774968,
-0.1996321678,
-0.0951335654,
-0.1437036842,
0.0538111962,
0.653909564,
0.0927327722,
-0.016800072,
0.0756470934,
-0.1002285406,
-0.3448368311,
-0.0425327159,
-0.1009554341,
0.2062197328,
0.2147901356,
0.0556404777,
0.2983597219,
0.1459521502,
-0.0644702911,
-0.4424965978,
0.1017011777,
-0.0400518253,
0.1395645589,
-0.145143196,
-0.1923438162,
-0.3297647238,
-0.0537859611,
-0.2079762369,
0.0183238313,
0.1633132249,
0.0683607757,
-0.2214221507,
-0.0204832722,
0.0140843056,
-0.075884521,
-0.0172140431,
0.1093305945,
-0.1040251106,
0.2656517625,
-0.0951162279,
0.1168940216,
-0.0267393459,
0.2531200051,
0.4326063693,
0.2400517166,
-0.0466214195,
0.367023617,
0.0848606005,
-0.3270231187,
0.0078404322,
-0.172658667,
0.0181292761,
-0.0838742778,
-0.0163266249,
0.1145247817,
0.2768396139,
-0.1085973084,
-0.0847191364,
0.1411861628,
-0.1772004515,
0.2762841582,
-0.1619747579,
0.2004606873,
0.2599629462,
-0.1093928516,
0.1251222938,
-0.2127112299,
0.2158810794,
-0.1297985166,
0.2343143374,
0.007142223,
-0.2617177367,
0.0677190349,
0.4220539927,
0.0015310015,
-0.0035239446,
0.009774005,
-0.1586676836,
0.1657464355,
-0.0130180242,
0.595718503,
0.352842629,
0.2059556991,
-0.1638759673,
-0.0582683198,
-0.2622588873,
-0.1369839609,
0.2124615908,
0.0951014385,
0.0298523773,
0.0572962686,
0.0261101257,
-0.0664390028,
-0.1765725613,
-0.2433390021,
0.2082311809,
0.3464297354,
-0.51031214,
-0.0029065625,
-0.3452727795,
-0.2318637222,
-0.3185971677,
-0.0987910777,
-0.2351591289,
-0.4590440094,
0.0904379636,
-0.0774915814,
-0.1903930455,
0.1450763792,
-0.0375638828,
0.0079173651,
-0.0910730362,
0.1520518214,
-0.0975839868,
-0.4702498615,
-0.2045714557,
0.1811123341,
0.0074800067,
0.0469533242,
0.4813601077,
-0.1431986392,
-0.1245555803,
-0.0785720423,
-0.2953169942,
0.0040264316,
-0.2730659246,
0.1366041899,
0.1816231608,
0.103859432,
0.0084615927,
-0.1486845016,
0.2930162251,
-0.0715196803,
0.0246398468,
0.1069570333,
0.0452330634,
-0.2920732796,
-0.2664697468,
-0.4750192463,
-0.4745301306,
-0.1774818748,
0.0669479594,
-0.075908266,
-0.0335285738,
0.5198025703,
0.0684420913,
0.0656812266,
0.0931449234,
0.023252707,
-0.3467434645,
-0.0935407355,
0.2490479201,
-0.179091692,
-0.210245356,
0.1960575432,
0.1093095243,
0.1175282896,
-0.20393309,
-0.3139223158,
0.0728625953,
-0.0576012135,
0.058944717,
0.0813103691,
0.1948962212,
0.2138828039,
0.2671021223,
-0.1480035037,
-0.3270511925,
-0.3317118883,
0.1381734908,
-0.0719676912,
0.1306350976,
-0.1062558591,
0.294085592,
-0.152083829,
0.326508671,
0.0217723008,
-0.2126558274,
0.191888988,
-0.0478476658,
0.5207321048,
0.068380706,
-0.4193751216,
0.0658852011,
-0.1191071793,
0.0269460715,
0.1760428697,
-0.3196389079,
0.0379566401,
-0.1479681134,
0.3946042061,
-0.2478049397,
-0.0996567011,
-0.1006427184,
-0.1501683444,
0.0405269749,
0.1169003174,
-0.0409751162,
-0.2084082365,
-0.1915850341,
-0.2512801886,
0.0578238554,
-0.0954614133,
-0.087551482,
-0.533575058,
-0.2334993929,
-0.1649958491,
0.2940982878,
0.1574513167,
0.3346639276,
-0.1785171777,
0.2454715222,
0.0477584898,
0.2458998859,
0.6149811745,
-0.4832390547,
0.154702425,
0.2263719141,
0.3931272328,
-0.1780015528,
-0.1272553653,
-0.1692574769,
0.2947262228,
-0.2957772911,
0.0259887744,
-0.1555344164,
-0.0910770446,
0.2631006241,
0.1554057747,
-0.1223803461,
-0.3149805069,
-0.2309516966,
-0.1793929189,
-0.1272854507,
-0.0533969514,
0.0635773465,
0.1866380721,
-0.3784870207,
0.0986478776,
0.1408236176,
0.0735073909,
0.2715045512,
0.1186348274,
0.2232429981,
0.0302767064,
0.197361365,
0.317402184,
0.2587257028,
0.5099822879,
0.5483981967,
-0.3618466854,
-0.5113294125,
-0.1194789112,
-0.1147691086,
0.2007077336,
0.0650010705,
-0.1422151178,
0.1660957336,
-0.0506398827,
0.185458824,
0.0961978808,
0.0223078672,
0.4922952354,
0.1605764627,
-0.4898479283,
-0.5388931632,
0.2713592649,
0.0392414108,
-0.0478406474,
0.1281552166,
0.0797321871,
-0.3407573402,
0.2059525549,
-0.1559936553,
0.6908729672,
0.0837977454,
-0.0510304905,
-0.0170310941,
-0.2261731625,
0.7132234573,
-0.1187111512,
0.0007714578,
-0.3614293039,
-0.3074660599,
-0.0622299947,
-0.0221186746,
0.0234751571,
0.4506933987,
-0.3085790277,
0.3788379431,
-0.3285133839,
0.2046867758,
0.028750021,
-0.0740614757,
0.2494399399,
-0.1323137879,
-0.0824863017,
0.2010339946,
-0.1576190293,
-0.1343754977,
-0.1674345434,
0.0419054106,
-0.2318485528,
-0.2680606246,
-0.2262603641,
0.08226078,
-0.2538248897,
0.1415783167,
-0.0856540874,
-0.0167195015,
0.2168119252,
0.2025102079,
0.4863218665,
0.0789240599,
-0.1482862979,
0.1838956922,
0.0421487242,
0.1370235533,
0.0343489051,
0.0537468866,
0.4150622785,
0.1160496846,
-0.2699809074,
0.1285305768,
-0.0795674771,
0.1759498864,
-0.0870310813,
0.0838943273,
-0.0983974338,
-0.3653748333,
0.0326472521,
-0.0770386755,
0.2287106365,
-0.2537733614,
0.1773460358,
0.0519831851,
-0.0466800705,
0.2120788842,
-0.2636915445,
-0.1465975195,
-0.0457466282,
0.1892329752,
0.0259641036,
0.1933565587,
0.2472447455,
0.0259730872,
-0.3513919413,
-0.2565112412,
0.038952101,
0.0239994247,
-0.6778352261,
0.2459824234,
0.2533547282,
0.0759458542,
-0.057189174,
0.2232685387,
-0.0158301294,
0.0710849985,
0.0610140152,
-0.5274376869,
-0.2186500877,
-0.00467043,
-0.1847544909,
0.1169297621,
0.2478654385,
0.4110050797,
0.1337576956,
0.0714501962,
-0.3641543388,
-0.020954119,
-0.172394067,
0.2980129123,
0.1525106579,
0.0199611541,
0.2820312381,
-0.1788062751,
0.218216911,
0.0007437372,
0.007750046,
-0.2498184741,
-0.1872092783,
0.1124106348,
0.1352093369,
-0.1972157359,
-0.0634114593,
-0.1643812805,
0.0578369349,
-0.0719840601,
-0.0172511172,
0.3105739653,
0.0032078198,
0.3735309839,
-0.1509219557,
0.2327846885,
-0.0463298075,
0.2182779461,
0.0127172945,
-0.114001587,
0.0742451549,
0.1729656607,
-0.1127646267,
0.0194780901,
-0.1750794947,
0.0847947747,
-0.1170051619,
-0.11792963,
0.1342932731,
-0.568980515,
0.2959564924,
0.193638131,
0.449362725,
0.2781369686,
-0.1868256927,
-0.0707662776,
0.3826093972,
0.307926774,
-0.3656698763,
-0.1683599651,
0.1630016863,
0.1655610651,
0.0003906599,
0.0756075159,
0.060725417,
-0.0310598556,
0.0448926538,
0.0080858618,
0.4554387927,
-0.4376546144,
0.2349053174,
0.5269825459,
-0.0619708523,
0.0999840051,
0.4967942238,
0.1731765717,
0.0635329187,
0.5881978869,
0.0346502028,
0.1773207486,
0.1874702275,
-0.1379599422,
-0.0754204541,
-0.172217235,
0.1809182614,
0.0969726592,
0.0188562367,
-0.0428314172,
-0.0954477713,
0.3011343777,
-0.3839455247,
0.0041103126,
-0.0057690036,
0.1111971214,
-0.2255034149,
-0.1060157418,
-0.1562631726,
-0.0987095013,
-0.1664459705,
-0.1973160356,
0.0140280882,
-0.1700504124,
0.3556354642,
-0.0742809027,
-0.1959641278,
-0.4565812349,
-0.186778456,
0.305419594,
0.065081425,
-0.2473097742,
0.0163199063,
0.2487559468,
-0.1455008835,
0.3213778436,
0.086239703,
0.6039388776,
0.1713752747,
0.0897912681,
-0.3410916328,
-0.0972951949,
-0.088580206,
0.1516636312,
0.215568006,
0.0459204242,
-0.0368339717,
0.3922656178,
0.2018781453,
-0.1707493216,
0.3085803986,
0.3357422054,
0.2227042168,
-0.170619756,
-0.0630670115,
-0.1339656562,
-0.2573547959,
-0.3266397119,
0.1013188735,
-0.2697949111,
0.1159574091,
0.4622558057,
-0.0415135659,
0.0840901211,
-0.2942293584,
0.1462800503,
0.1317104101,
0.3118901849,
0.2739442885,
-0.0476244502,
-0.3456508517,
0.1615712494,
-0.5773795247,
0.129460454,
-0.1186997294,
-0.1146923974,
-0.0334540457,
-0.1582231671,
0.1593217105,
0.020614991,
0.3829501867,
0.1888182312,
-0.0159400646,
-0.1086774766,
-0.1195141748,
-0.1931985319,
-0.0825988874,
-0.1219008714,
0.2010165602,
-0.3226899803,
-0.0618356615,
-0.1457770467,
0.038975928,
-0.3498083949,
0.0937143192,
0.1062969789,
-0.0201894138,
0.3603177369,
0.1459479332,
0.7699726224,
0.048815161,
0.1150308847,
-0.0913875476,
-0.353649199,
-0.4042430222,
0.3688688576,
0.3255911171,
0.2565357983,
-0.1063772365,
0.0097641386,
-0.1644826233,
0.0945067257,
-0.04354655,
0.3211485744,
-0.2544759512,
0.2145047933,
-0.0240108576,
0.0171401687,
0.0991312191,
-0.0556259044,
-0.0026464916,
0.3410544395,
-0.2067562938,
-0.6047096252,
0.6198091507,
-0.212931633,
-0.2395552248,
0.1537303925,
0.1545257866,
0.2213794589,
-0.2187473923,
-0.5100530386,
0.0247500949,
0.3773825169,
0.0190039705,
-0.4423446655,
-0.0037064492,
-0.1927573532,
0.051444225,
0.113560237,
0.3062537014,
0.1166288406,
-0.1932859123,
0.1991046965,
-0.2543466091
]
|
https://github.com/huggingface/datasets/issues/1989 | Question/problem with dataset labels | Hi,
Just in case you want to use tsv directly, you can use the separator argument while loading the dataset.
```python
d = load_dataset("csv",data_files=['test.csv'],sep="\t")
```
Additionally, I don't face the issues with the following csv (same as the one you provided):
```sh
link1,text1,info1,info2,info3,info4,info5,link2,text2,text3
crawl-data/CC-MAIN-2017-47/segments/1510934806225.78/wet/CC-MAIN-20171120203833-20171120223833-00571.warc.wet.gz,Rose Blakey is an aspiring journalist. She is desperate to escape the from the small Australian town in which she lives. Rejection after rejection mean she is stuck in what she sees as a dead,"('Rose', '', 'Blakey')",journalist,F,38,journalist,https://www.netgalley.com/catalog/book/121872,_ is desperate to escape the from the small Australian town in which _ lives. Rejection after rejection mean _ is stuck in what _ sees as a dead-end waitressing job., She is desperate to escape the from the small Australian town in which she lives. Rejection after rejection mean she is stuck in what she sees as a dead-end waitressing job.
```
Output after loading:
```sh
{'link1': 'crawl-data/CC-MAIN-2017-47/segments/1510934806225.78/wet/CC-MAIN-20171120203833-20171120223833-00571.warc.wet.gz', 'text1': 'Rose Blakey is an aspiring journalist. She is desperate to escape the from the small Australian town in which she lives. Rejection after rejection mean she is stuck in what she sees as a dead', 'info1': "('Rose', '', 'Blakey')", 'info2': 'journalist', 'info3': 'F', 'info4': 38, 'info5': 'journalist', 'link2': 'https://www.netgalley.com/catalog/book/121872', 'text2': '_ is desperate to escape the from the small Australian town in which _ lives. Rejection after rejection mean _ is stuck in what _ sees as a dead-end waitressing job.', 'text3': ' She is desperate to escape the from the small Australian town in which she lives. Rejection after rejection mean she is stuck in what she sees as a dead-end waitressing job.'}
```
Can you check once if the tsv works for you directly using the separator argument? The conversion from tsv to csv could create issues, I'm only guessing though. | Hi, I'm using a dataset with two labels "nurse" and "not nurse". For whatever reason (that I don't understand), I get an error that I think comes from the datasets package (using csv). Everything works fine if the labels are "nurse" and "surgeon".
This is the trace I get:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: not nurse
```
Any ideas how to fix this? For now, I'll probably make them numeric. | 292 | Question/problem with dataset labels
Hi, I'm using a dataset with two labels "nurse" and "not nurse". For whatever reason (that I don't understand), I get an error that I think comes from the datasets package (using csv). Everything works fine if the labels are "nurse" and "surgeon".
This is the trace I get:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: not nurse
```
Any ideas how to fix this? For now, I'll probably make them numeric.
Hi,
Just in case you want to use tsv directly, you can use the separator argument while loading the dataset.
```python
d = load_dataset("csv",data_files=['test.csv'],sep="\t")
```
Additionally, I don't face the issues with the following csv (same as the one you provided):
```sh
link1,text1,info1,info2,info3,info4,info5,link2,text2,text3
crawl-data/CC-MAIN-2017-47/segments/1510934806225.78/wet/CC-MAIN-20171120203833-20171120223833-00571.warc.wet.gz,Rose Blakey is an aspiring journalist. She is desperate to escape the from the small Australian town in which she lives. Rejection after rejection mean she is stuck in what she sees as a dead,"('Rose', '', 'Blakey')",journalist,F,38,journalist,https://www.netgalley.com/catalog/book/121872,_ is desperate to escape the from the small Australian town in which _ lives. Rejection after rejection mean _ is stuck in what _ sees as a dead-end waitressing job., She is desperate to escape the from the small Australian town in which she lives. Rejection after rejection mean she is stuck in what she sees as a dead-end waitressing job.
```
Output after loading:
```sh
{'link1': 'crawl-data/CC-MAIN-2017-47/segments/1510934806225.78/wet/CC-MAIN-20171120203833-20171120223833-00571.warc.wet.gz', 'text1': 'Rose Blakey is an aspiring journalist. She is desperate to escape the from the small Australian town in which she lives. Rejection after rejection mean she is stuck in what she sees as a dead', 'info1': "('Rose', '', 'Blakey')", 'info2': 'journalist', 'info3': 'F', 'info4': 38, 'info5': 'journalist', 'link2': 'https://www.netgalley.com/catalog/book/121872', 'text2': '_ is desperate to escape the from the small Australian town in which _ lives. Rejection after rejection mean _ is stuck in what _ sees as a dead-end waitressing job.', 'text3': ' She is desperate to escape the from the small Australian town in which she lives. Rejection after rejection mean she is stuck in what she sees as a dead-end waitressing job.'}
```
Can you check once if the tsv works for you directly using the separator argument? The conversion from tsv to csv could create issues, I'm only guessing though. | [
0.1789148301,
-0.0573230609,
0.0051793531,
0.1343671232,
0.4113537371,
0.3502124548,
0.6419945955,
0.1637074798,
-0.1613179296,
0.0723498836,
0.1788543165,
0.0638753474,
-0.0607729964,
0.030525703,
-0.130230993,
-0.1193704456,
0.0890837312,
0.1734791994,
0.1816426963,
-0.04446676,
-0.2867425382,
-0.0398259945,
-0.156679973,
0.3662885129,
-0.297205627,
-0.3766838312,
0.2105430812,
-0.1205282882,
-0.1131331921,
-0.4633954763,
0.2718095183,
0.0861200094,
0.0026800728,
0.4034268558,
-0.0001030963,
0.058756806,
0.2967477739,
0.1559889019,
-0.3984076679,
-0.4321287572,
-0.1893597841,
-0.0234080795,
0.0345638432,
-0.3044767976,
0.0979833305,
-0.2846682072,
-0.0905410498,
-0.2756658196,
0.1046864912,
0.4386059344,
0.3266399205,
0.2373396754,
-0.2065001428,
-0.2293031216,
0.2311526835,
0.1250885725,
-0.0305089504,
0.0494753644,
-0.1344386041,
-0.0064582187,
0.4515704513,
0.460914433,
-0.2723956704,
0.2647709846,
0.1353648007,
0.1463635266,
0.1377247274,
-0.3910481632,
0.2763096094,
0.1961432248,
0.7452604771,
-0.2964998186,
-0.2943911254,
-0.1697053015,
0.1373766065,
-0.4167195559,
0.1813390404,
0.0700832456,
0.0063756779,
-0.0470148437,
-0.0331627578,
0.0610270314,
0.0807417855,
0.0881804153,
-0.0580748878,
0.0383284204,
-0.0986412764,
0.3288168907,
0.1125301495,
-0.0747507066,
0.2975301445,
-0.0059941988,
-0.0885701999,
0.0415449142,
-0.4325241745,
-0.0296084415,
-0.0368810184,
0.0516759381,
-0.1181376055,
-0.038740769,
0.0183869507,
-0.3749563098,
-0.1474356651,
0.3403449953,
0.2151344717,
0.2662764788,
0.2706995606,
0.5616863966,
0.0911723822,
-0.0761627182,
0.0308040548,
-0.0558074787,
-0.0015739995,
-0.4626432061,
0.2433210015,
0.0989312604,
0.2934703231,
-0.2398585975,
-0.5455213189,
0.1924421936,
-0.0281014666,
0.0940956026,
0.1541178226,
0.3049503565,
0.0221220106,
0.2065178901,
0.0793469325,
0.193608433,
-0.0091703041,
-0.1172054783,
-0.2069241703,
0.0976080745,
-0.2002791017,
-0.150106892,
0.0502697304,
-0.0720530748,
0.1124490499,
0.0640597194,
-0.0010049042,
-0.0760566816,
-0.0163034722,
-0.3864641488,
-0.012748206,
0.3588934243,
-0.1032633781,
0.420350641,
0.2520543337,
-0.4185501039,
0.0596993491,
0.1802113652,
-0.3688521683,
-0.0338135473,
-0.2121574432,
0.2862125635,
-0.1030441746,
-0.187981084,
-0.1200913265,
0.0468237363,
0.4193198979,
-0.2145542502,
0.1575898081,
-0.3543840945,
-0.3093222678,
-0.2777702212,
-0.0663038418,
0.2214321792,
-0.6722466946,
0.004389429,
-0.1475467384,
-0.0291129835,
0.0528065376,
0.2960194051,
-0.0810041502,
0.2062916607,
-0.0705919117,
0.0303497873,
0.1395514011,
-0.390691489,
-0.1104637682,
0.1035439447,
-0.1420033723,
-0.2442642748,
0.0704807937,
-0.0403395221,
0.0917313397,
-0.0916260481,
0.0582318865,
-0.1318774968,
-0.1996321678,
-0.0951335654,
-0.1437036842,
0.0538111962,
0.653909564,
0.0927327722,
-0.016800072,
0.0756470934,
-0.1002285406,
-0.3448368311,
-0.0425327159,
-0.1009554341,
0.2062197328,
0.2147901356,
0.0556404777,
0.2983597219,
0.1459521502,
-0.0644702911,
-0.4424965978,
0.1017011777,
-0.0400518253,
0.1395645589,
-0.145143196,
-0.1923438162,
-0.3297647238,
-0.0537859611,
-0.2079762369,
0.0183238313,
0.1633132249,
0.0683607757,
-0.2214221507,
-0.0204832722,
0.0140843056,
-0.075884521,
-0.0172140431,
0.1093305945,
-0.1040251106,
0.2656517625,
-0.0951162279,
0.1168940216,
-0.0267393459,
0.2531200051,
0.4326063693,
0.2400517166,
-0.0466214195,
0.367023617,
0.0848606005,
-0.3270231187,
0.0078404322,
-0.172658667,
0.0181292761,
-0.0838742778,
-0.0163266249,
0.1145247817,
0.2768396139,
-0.1085973084,
-0.0847191364,
0.1411861628,
-0.1772004515,
0.2762841582,
-0.1619747579,
0.2004606873,
0.2599629462,
-0.1093928516,
0.1251222938,
-0.2127112299,
0.2158810794,
-0.1297985166,
0.2343143374,
0.007142223,
-0.2617177367,
0.0677190349,
0.4220539927,
0.0015310015,
-0.0035239446,
0.009774005,
-0.1586676836,
0.1657464355,
-0.0130180242,
0.595718503,
0.352842629,
0.2059556991,
-0.1638759673,
-0.0582683198,
-0.2622588873,
-0.1369839609,
0.2124615908,
0.0951014385,
0.0298523773,
0.0572962686,
0.0261101257,
-0.0664390028,
-0.1765725613,
-0.2433390021,
0.2082311809,
0.3464297354,
-0.51031214,
-0.0029065625,
-0.3452727795,
-0.2318637222,
-0.3185971677,
-0.0987910777,
-0.2351591289,
-0.4590440094,
0.0904379636,
-0.0774915814,
-0.1903930455,
0.1450763792,
-0.0375638828,
0.0079173651,
-0.0910730362,
0.1520518214,
-0.0975839868,
-0.4702498615,
-0.2045714557,
0.1811123341,
0.0074800067,
0.0469533242,
0.4813601077,
-0.1431986392,
-0.1245555803,
-0.0785720423,
-0.2953169942,
0.0040264316,
-0.2730659246,
0.1366041899,
0.1816231608,
0.103859432,
0.0084615927,
-0.1486845016,
0.2930162251,
-0.0715196803,
0.0246398468,
0.1069570333,
0.0452330634,
-0.2920732796,
-0.2664697468,
-0.4750192463,
-0.4745301306,
-0.1774818748,
0.0669479594,
-0.075908266,
-0.0335285738,
0.5198025703,
0.0684420913,
0.0656812266,
0.0931449234,
0.023252707,
-0.3467434645,
-0.0935407355,
0.2490479201,
-0.179091692,
-0.210245356,
0.1960575432,
0.1093095243,
0.1175282896,
-0.20393309,
-0.3139223158,
0.0728625953,
-0.0576012135,
0.058944717,
0.0813103691,
0.1948962212,
0.2138828039,
0.2671021223,
-0.1480035037,
-0.3270511925,
-0.3317118883,
0.1381734908,
-0.0719676912,
0.1306350976,
-0.1062558591,
0.294085592,
-0.152083829,
0.326508671,
0.0217723008,
-0.2126558274,
0.191888988,
-0.0478476658,
0.5207321048,
0.068380706,
-0.4193751216,
0.0658852011,
-0.1191071793,
0.0269460715,
0.1760428697,
-0.3196389079,
0.0379566401,
-0.1479681134,
0.3946042061,
-0.2478049397,
-0.0996567011,
-0.1006427184,
-0.1501683444,
0.0405269749,
0.1169003174,
-0.0409751162,
-0.2084082365,
-0.1915850341,
-0.2512801886,
0.0578238554,
-0.0954614133,
-0.087551482,
-0.533575058,
-0.2334993929,
-0.1649958491,
0.2940982878,
0.1574513167,
0.3346639276,
-0.1785171777,
0.2454715222,
0.0477584898,
0.2458998859,
0.6149811745,
-0.4832390547,
0.154702425,
0.2263719141,
0.3931272328,
-0.1780015528,
-0.1272553653,
-0.1692574769,
0.2947262228,
-0.2957772911,
0.0259887744,
-0.1555344164,
-0.0910770446,
0.2631006241,
0.1554057747,
-0.1223803461,
-0.3149805069,
-0.2309516966,
-0.1793929189,
-0.1272854507,
-0.0533969514,
0.0635773465,
0.1866380721,
-0.3784870207,
0.0986478776,
0.1408236176,
0.0735073909,
0.2715045512,
0.1186348274,
0.2232429981,
0.0302767064,
0.197361365,
0.317402184,
0.2587257028,
0.5099822879,
0.5483981967,
-0.3618466854,
-0.5113294125,
-0.1194789112,
-0.1147691086,
0.2007077336,
0.0650010705,
-0.1422151178,
0.1660957336,
-0.0506398827,
0.185458824,
0.0961978808,
0.0223078672,
0.4922952354,
0.1605764627,
-0.4898479283,
-0.5388931632,
0.2713592649,
0.0392414108,
-0.0478406474,
0.1281552166,
0.0797321871,
-0.3407573402,
0.2059525549,
-0.1559936553,
0.6908729672,
0.0837977454,
-0.0510304905,
-0.0170310941,
-0.2261731625,
0.7132234573,
-0.1187111512,
0.0007714578,
-0.3614293039,
-0.3074660599,
-0.0622299947,
-0.0221186746,
0.0234751571,
0.4506933987,
-0.3085790277,
0.3788379431,
-0.3285133839,
0.2046867758,
0.028750021,
-0.0740614757,
0.2494399399,
-0.1323137879,
-0.0824863017,
0.2010339946,
-0.1576190293,
-0.1343754977,
-0.1674345434,
0.0419054106,
-0.2318485528,
-0.2680606246,
-0.2262603641,
0.08226078,
-0.2538248897,
0.1415783167,
-0.0856540874,
-0.0167195015,
0.2168119252,
0.2025102079,
0.4863218665,
0.0789240599,
-0.1482862979,
0.1838956922,
0.0421487242,
0.1370235533,
0.0343489051,
0.0537468866,
0.4150622785,
0.1160496846,
-0.2699809074,
0.1285305768,
-0.0795674771,
0.1759498864,
-0.0870310813,
0.0838943273,
-0.0983974338,
-0.3653748333,
0.0326472521,
-0.0770386755,
0.2287106365,
-0.2537733614,
0.1773460358,
0.0519831851,
-0.0466800705,
0.2120788842,
-0.2636915445,
-0.1465975195,
-0.0457466282,
0.1892329752,
0.0259641036,
0.1933565587,
0.2472447455,
0.0259730872,
-0.3513919413,
-0.2565112412,
0.038952101,
0.0239994247,
-0.6778352261,
0.2459824234,
0.2533547282,
0.0759458542,
-0.057189174,
0.2232685387,
-0.0158301294,
0.0710849985,
0.0610140152,
-0.5274376869,
-0.2186500877,
-0.00467043,
-0.1847544909,
0.1169297621,
0.2478654385,
0.4110050797,
0.1337576956,
0.0714501962,
-0.3641543388,
-0.020954119,
-0.172394067,
0.2980129123,
0.1525106579,
0.0199611541,
0.2820312381,
-0.1788062751,
0.218216911,
0.0007437372,
0.007750046,
-0.2498184741,
-0.1872092783,
0.1124106348,
0.1352093369,
-0.1972157359,
-0.0634114593,
-0.1643812805,
0.0578369349,
-0.0719840601,
-0.0172511172,
0.3105739653,
0.0032078198,
0.3735309839,
-0.1509219557,
0.2327846885,
-0.0463298075,
0.2182779461,
0.0127172945,
-0.114001587,
0.0742451549,
0.1729656607,
-0.1127646267,
0.0194780901,
-0.1750794947,
0.0847947747,
-0.1170051619,
-0.11792963,
0.1342932731,
-0.568980515,
0.2959564924,
0.193638131,
0.449362725,
0.2781369686,
-0.1868256927,
-0.0707662776,
0.3826093972,
0.307926774,
-0.3656698763,
-0.1683599651,
0.1630016863,
0.1655610651,
0.0003906599,
0.0756075159,
0.060725417,
-0.0310598556,
0.0448926538,
0.0080858618,
0.4554387927,
-0.4376546144,
0.2349053174,
0.5269825459,
-0.0619708523,
0.0999840051,
0.4967942238,
0.1731765717,
0.0635329187,
0.5881978869,
0.0346502028,
0.1773207486,
0.1874702275,
-0.1379599422,
-0.0754204541,
-0.172217235,
0.1809182614,
0.0969726592,
0.0188562367,
-0.0428314172,
-0.0954477713,
0.3011343777,
-0.3839455247,
0.0041103126,
-0.0057690036,
0.1111971214,
-0.2255034149,
-0.1060157418,
-0.1562631726,
-0.0987095013,
-0.1664459705,
-0.1973160356,
0.0140280882,
-0.1700504124,
0.3556354642,
-0.0742809027,
-0.1959641278,
-0.4565812349,
-0.186778456,
0.305419594,
0.065081425,
-0.2473097742,
0.0163199063,
0.2487559468,
-0.1455008835,
0.3213778436,
0.086239703,
0.6039388776,
0.1713752747,
0.0897912681,
-0.3410916328,
-0.0972951949,
-0.088580206,
0.1516636312,
0.215568006,
0.0459204242,
-0.0368339717,
0.3922656178,
0.2018781453,
-0.1707493216,
0.3085803986,
0.3357422054,
0.2227042168,
-0.170619756,
-0.0630670115,
-0.1339656562,
-0.2573547959,
-0.3266397119,
0.1013188735,
-0.2697949111,
0.1159574091,
0.4622558057,
-0.0415135659,
0.0840901211,
-0.2942293584,
0.1462800503,
0.1317104101,
0.3118901849,
0.2739442885,
-0.0476244502,
-0.3456508517,
0.1615712494,
-0.5773795247,
0.129460454,
-0.1186997294,
-0.1146923974,
-0.0334540457,
-0.1582231671,
0.1593217105,
0.020614991,
0.3829501867,
0.1888182312,
-0.0159400646,
-0.1086774766,
-0.1195141748,
-0.1931985319,
-0.0825988874,
-0.1219008714,
0.2010165602,
-0.3226899803,
-0.0618356615,
-0.1457770467,
0.038975928,
-0.3498083949,
0.0937143192,
0.1062969789,
-0.0201894138,
0.3603177369,
0.1459479332,
0.7699726224,
0.048815161,
0.1150308847,
-0.0913875476,
-0.353649199,
-0.4042430222,
0.3688688576,
0.3255911171,
0.2565357983,
-0.1063772365,
0.0097641386,
-0.1644826233,
0.0945067257,
-0.04354655,
0.3211485744,
-0.2544759512,
0.2145047933,
-0.0240108576,
0.0171401687,
0.0991312191,
-0.0556259044,
-0.0026464916,
0.3410544395,
-0.2067562938,
-0.6047096252,
0.6198091507,
-0.212931633,
-0.2395552248,
0.1537303925,
0.1545257866,
0.2213794589,
-0.2187473923,
-0.5100530386,
0.0247500949,
0.3773825169,
0.0190039705,
-0.4423446655,
-0.0037064492,
-0.1927573532,
0.051444225,
0.113560237,
0.3062537014,
0.1166288406,
-0.1932859123,
0.1991046965,
-0.2543466091
]
|
https://github.com/huggingface/datasets/issues/1989 | Question/problem with dataset labels | thanks for the tip. very strange :/ I'll check my datasets version as well.
I will have more similar experiments soon so I'll let you know if I manage to get rid of this. | Hi, I'm using a dataset with two labels "nurse" and "not nurse". For whatever reason (that I don't understand), I get an error that I think comes from the datasets package (using csv). Everything works fine if the labels are "nurse" and "surgeon".
This is the trace I get:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: not nurse
```
Any ideas how to fix this? For now, I'll probably make them numeric. | 34 | Question/problem with dataset labels
Hi, I'm using a dataset with two labels "nurse" and "not nurse". For whatever reason (that I don't understand), I get an error that I think comes from the datasets package (using csv). Everything works fine if the labels are "nurse" and "surgeon".
This is the trace I get:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: not nurse
```
Any ideas how to fix this? For now, I'll probably make them numeric.
thanks for the tip. very strange :/ I'll check my datasets version as well.
I will have more similar experiments soon so I'll let you know if I manage to get rid of this. | [
0.1789148301,
-0.0573230609,
0.0051793531,
0.1343671232,
0.4113537371,
0.3502124548,
0.6419945955,
0.1637074798,
-0.1613179296,
0.0723498836,
0.1788543165,
0.0638753474,
-0.0607729964,
0.030525703,
-0.130230993,
-0.1193704456,
0.0890837312,
0.1734791994,
0.1816426963,
-0.04446676,
-0.2867425382,
-0.0398259945,
-0.156679973,
0.3662885129,
-0.297205627,
-0.3766838312,
0.2105430812,
-0.1205282882,
-0.1131331921,
-0.4633954763,
0.2718095183,
0.0861200094,
0.0026800728,
0.4034268558,
-0.0001030963,
0.058756806,
0.2967477739,
0.1559889019,
-0.3984076679,
-0.4321287572,
-0.1893597841,
-0.0234080795,
0.0345638432,
-0.3044767976,
0.0979833305,
-0.2846682072,
-0.0905410498,
-0.2756658196,
0.1046864912,
0.4386059344,
0.3266399205,
0.2373396754,
-0.2065001428,
-0.2293031216,
0.2311526835,
0.1250885725,
-0.0305089504,
0.0494753644,
-0.1344386041,
-0.0064582187,
0.4515704513,
0.460914433,
-0.2723956704,
0.2647709846,
0.1353648007,
0.1463635266,
0.1377247274,
-0.3910481632,
0.2763096094,
0.1961432248,
0.7452604771,
-0.2964998186,
-0.2943911254,
-0.1697053015,
0.1373766065,
-0.4167195559,
0.1813390404,
0.0700832456,
0.0063756779,
-0.0470148437,
-0.0331627578,
0.0610270314,
0.0807417855,
0.0881804153,
-0.0580748878,
0.0383284204,
-0.0986412764,
0.3288168907,
0.1125301495,
-0.0747507066,
0.2975301445,
-0.0059941988,
-0.0885701999,
0.0415449142,
-0.4325241745,
-0.0296084415,
-0.0368810184,
0.0516759381,
-0.1181376055,
-0.038740769,
0.0183869507,
-0.3749563098,
-0.1474356651,
0.3403449953,
0.2151344717,
0.2662764788,
0.2706995606,
0.5616863966,
0.0911723822,
-0.0761627182,
0.0308040548,
-0.0558074787,
-0.0015739995,
-0.4626432061,
0.2433210015,
0.0989312604,
0.2934703231,
-0.2398585975,
-0.5455213189,
0.1924421936,
-0.0281014666,
0.0940956026,
0.1541178226,
0.3049503565,
0.0221220106,
0.2065178901,
0.0793469325,
0.193608433,
-0.0091703041,
-0.1172054783,
-0.2069241703,
0.0976080745,
-0.2002791017,
-0.150106892,
0.0502697304,
-0.0720530748,
0.1124490499,
0.0640597194,
-0.0010049042,
-0.0760566816,
-0.0163034722,
-0.3864641488,
-0.012748206,
0.3588934243,
-0.1032633781,
0.420350641,
0.2520543337,
-0.4185501039,
0.0596993491,
0.1802113652,
-0.3688521683,
-0.0338135473,
-0.2121574432,
0.2862125635,
-0.1030441746,
-0.187981084,
-0.1200913265,
0.0468237363,
0.4193198979,
-0.2145542502,
0.1575898081,
-0.3543840945,
-0.3093222678,
-0.2777702212,
-0.0663038418,
0.2214321792,
-0.6722466946,
0.004389429,
-0.1475467384,
-0.0291129835,
0.0528065376,
0.2960194051,
-0.0810041502,
0.2062916607,
-0.0705919117,
0.0303497873,
0.1395514011,
-0.390691489,
-0.1104637682,
0.1035439447,
-0.1420033723,
-0.2442642748,
0.0704807937,
-0.0403395221,
0.0917313397,
-0.0916260481,
0.0582318865,
-0.1318774968,
-0.1996321678,
-0.0951335654,
-0.1437036842,
0.0538111962,
0.653909564,
0.0927327722,
-0.016800072,
0.0756470934,
-0.1002285406,
-0.3448368311,
-0.0425327159,
-0.1009554341,
0.2062197328,
0.2147901356,
0.0556404777,
0.2983597219,
0.1459521502,
-0.0644702911,
-0.4424965978,
0.1017011777,
-0.0400518253,
0.1395645589,
-0.145143196,
-0.1923438162,
-0.3297647238,
-0.0537859611,
-0.2079762369,
0.0183238313,
0.1633132249,
0.0683607757,
-0.2214221507,
-0.0204832722,
0.0140843056,
-0.075884521,
-0.0172140431,
0.1093305945,
-0.1040251106,
0.2656517625,
-0.0951162279,
0.1168940216,
-0.0267393459,
0.2531200051,
0.4326063693,
0.2400517166,
-0.0466214195,
0.367023617,
0.0848606005,
-0.3270231187,
0.0078404322,
-0.172658667,
0.0181292761,
-0.0838742778,
-0.0163266249,
0.1145247817,
0.2768396139,
-0.1085973084,
-0.0847191364,
0.1411861628,
-0.1772004515,
0.2762841582,
-0.1619747579,
0.2004606873,
0.2599629462,
-0.1093928516,
0.1251222938,
-0.2127112299,
0.2158810794,
-0.1297985166,
0.2343143374,
0.007142223,
-0.2617177367,
0.0677190349,
0.4220539927,
0.0015310015,
-0.0035239446,
0.009774005,
-0.1586676836,
0.1657464355,
-0.0130180242,
0.595718503,
0.352842629,
0.2059556991,
-0.1638759673,
-0.0582683198,
-0.2622588873,
-0.1369839609,
0.2124615908,
0.0951014385,
0.0298523773,
0.0572962686,
0.0261101257,
-0.0664390028,
-0.1765725613,
-0.2433390021,
0.2082311809,
0.3464297354,
-0.51031214,
-0.0029065625,
-0.3452727795,
-0.2318637222,
-0.3185971677,
-0.0987910777,
-0.2351591289,
-0.4590440094,
0.0904379636,
-0.0774915814,
-0.1903930455,
0.1450763792,
-0.0375638828,
0.0079173651,
-0.0910730362,
0.1520518214,
-0.0975839868,
-0.4702498615,
-0.2045714557,
0.1811123341,
0.0074800067,
0.0469533242,
0.4813601077,
-0.1431986392,
-0.1245555803,
-0.0785720423,
-0.2953169942,
0.0040264316,
-0.2730659246,
0.1366041899,
0.1816231608,
0.103859432,
0.0084615927,
-0.1486845016,
0.2930162251,
-0.0715196803,
0.0246398468,
0.1069570333,
0.0452330634,
-0.2920732796,
-0.2664697468,
-0.4750192463,
-0.4745301306,
-0.1774818748,
0.0669479594,
-0.075908266,
-0.0335285738,
0.5198025703,
0.0684420913,
0.0656812266,
0.0931449234,
0.023252707,
-0.3467434645,
-0.0935407355,
0.2490479201,
-0.179091692,
-0.210245356,
0.1960575432,
0.1093095243,
0.1175282896,
-0.20393309,
-0.3139223158,
0.0728625953,
-0.0576012135,
0.058944717,
0.0813103691,
0.1948962212,
0.2138828039,
0.2671021223,
-0.1480035037,
-0.3270511925,
-0.3317118883,
0.1381734908,
-0.0719676912,
0.1306350976,
-0.1062558591,
0.294085592,
-0.152083829,
0.326508671,
0.0217723008,
-0.2126558274,
0.191888988,
-0.0478476658,
0.5207321048,
0.068380706,
-0.4193751216,
0.0658852011,
-0.1191071793,
0.0269460715,
0.1760428697,
-0.3196389079,
0.0379566401,
-0.1479681134,
0.3946042061,
-0.2478049397,
-0.0996567011,
-0.1006427184,
-0.1501683444,
0.0405269749,
0.1169003174,
-0.0409751162,
-0.2084082365,
-0.1915850341,
-0.2512801886,
0.0578238554,
-0.0954614133,
-0.087551482,
-0.533575058,
-0.2334993929,
-0.1649958491,
0.2940982878,
0.1574513167,
0.3346639276,
-0.1785171777,
0.2454715222,
0.0477584898,
0.2458998859,
0.6149811745,
-0.4832390547,
0.154702425,
0.2263719141,
0.3931272328,
-0.1780015528,
-0.1272553653,
-0.1692574769,
0.2947262228,
-0.2957772911,
0.0259887744,
-0.1555344164,
-0.0910770446,
0.2631006241,
0.1554057747,
-0.1223803461,
-0.3149805069,
-0.2309516966,
-0.1793929189,
-0.1272854507,
-0.0533969514,
0.0635773465,
0.1866380721,
-0.3784870207,
0.0986478776,
0.1408236176,
0.0735073909,
0.2715045512,
0.1186348274,
0.2232429981,
0.0302767064,
0.197361365,
0.317402184,
0.2587257028,
0.5099822879,
0.5483981967,
-0.3618466854,
-0.5113294125,
-0.1194789112,
-0.1147691086,
0.2007077336,
0.0650010705,
-0.1422151178,
0.1660957336,
-0.0506398827,
0.185458824,
0.0961978808,
0.0223078672,
0.4922952354,
0.1605764627,
-0.4898479283,
-0.5388931632,
0.2713592649,
0.0392414108,
-0.0478406474,
0.1281552166,
0.0797321871,
-0.3407573402,
0.2059525549,
-0.1559936553,
0.6908729672,
0.0837977454,
-0.0510304905,
-0.0170310941,
-0.2261731625,
0.7132234573,
-0.1187111512,
0.0007714578,
-0.3614293039,
-0.3074660599,
-0.0622299947,
-0.0221186746,
0.0234751571,
0.4506933987,
-0.3085790277,
0.3788379431,
-0.3285133839,
0.2046867758,
0.028750021,
-0.0740614757,
0.2494399399,
-0.1323137879,
-0.0824863017,
0.2010339946,
-0.1576190293,
-0.1343754977,
-0.1674345434,
0.0419054106,
-0.2318485528,
-0.2680606246,
-0.2262603641,
0.08226078,
-0.2538248897,
0.1415783167,
-0.0856540874,
-0.0167195015,
0.2168119252,
0.2025102079,
0.4863218665,
0.0789240599,
-0.1482862979,
0.1838956922,
0.0421487242,
0.1370235533,
0.0343489051,
0.0537468866,
0.4150622785,
0.1160496846,
-0.2699809074,
0.1285305768,
-0.0795674771,
0.1759498864,
-0.0870310813,
0.0838943273,
-0.0983974338,
-0.3653748333,
0.0326472521,
-0.0770386755,
0.2287106365,
-0.2537733614,
0.1773460358,
0.0519831851,
-0.0466800705,
0.2120788842,
-0.2636915445,
-0.1465975195,
-0.0457466282,
0.1892329752,
0.0259641036,
0.1933565587,
0.2472447455,
0.0259730872,
-0.3513919413,
-0.2565112412,
0.038952101,
0.0239994247,
-0.6778352261,
0.2459824234,
0.2533547282,
0.0759458542,
-0.057189174,
0.2232685387,
-0.0158301294,
0.0710849985,
0.0610140152,
-0.5274376869,
-0.2186500877,
-0.00467043,
-0.1847544909,
0.1169297621,
0.2478654385,
0.4110050797,
0.1337576956,
0.0714501962,
-0.3641543388,
-0.020954119,
-0.172394067,
0.2980129123,
0.1525106579,
0.0199611541,
0.2820312381,
-0.1788062751,
0.218216911,
0.0007437372,
0.007750046,
-0.2498184741,
-0.1872092783,
0.1124106348,
0.1352093369,
-0.1972157359,
-0.0634114593,
-0.1643812805,
0.0578369349,
-0.0719840601,
-0.0172511172,
0.3105739653,
0.0032078198,
0.3735309839,
-0.1509219557,
0.2327846885,
-0.0463298075,
0.2182779461,
0.0127172945,
-0.114001587,
0.0742451549,
0.1729656607,
-0.1127646267,
0.0194780901,
-0.1750794947,
0.0847947747,
-0.1170051619,
-0.11792963,
0.1342932731,
-0.568980515,
0.2959564924,
0.193638131,
0.449362725,
0.2781369686,
-0.1868256927,
-0.0707662776,
0.3826093972,
0.307926774,
-0.3656698763,
-0.1683599651,
0.1630016863,
0.1655610651,
0.0003906599,
0.0756075159,
0.060725417,
-0.0310598556,
0.0448926538,
0.0080858618,
0.4554387927,
-0.4376546144,
0.2349053174,
0.5269825459,
-0.0619708523,
0.0999840051,
0.4967942238,
0.1731765717,
0.0635329187,
0.5881978869,
0.0346502028,
0.1773207486,
0.1874702275,
-0.1379599422,
-0.0754204541,
-0.172217235,
0.1809182614,
0.0969726592,
0.0188562367,
-0.0428314172,
-0.0954477713,
0.3011343777,
-0.3839455247,
0.0041103126,
-0.0057690036,
0.1111971214,
-0.2255034149,
-0.1060157418,
-0.1562631726,
-0.0987095013,
-0.1664459705,
-0.1973160356,
0.0140280882,
-0.1700504124,
0.3556354642,
-0.0742809027,
-0.1959641278,
-0.4565812349,
-0.186778456,
0.305419594,
0.065081425,
-0.2473097742,
0.0163199063,
0.2487559468,
-0.1455008835,
0.3213778436,
0.086239703,
0.6039388776,
0.1713752747,
0.0897912681,
-0.3410916328,
-0.0972951949,
-0.088580206,
0.1516636312,
0.215568006,
0.0459204242,
-0.0368339717,
0.3922656178,
0.2018781453,
-0.1707493216,
0.3085803986,
0.3357422054,
0.2227042168,
-0.170619756,
-0.0630670115,
-0.1339656562,
-0.2573547959,
-0.3266397119,
0.1013188735,
-0.2697949111,
0.1159574091,
0.4622558057,
-0.0415135659,
0.0840901211,
-0.2942293584,
0.1462800503,
0.1317104101,
0.3118901849,
0.2739442885,
-0.0476244502,
-0.3456508517,
0.1615712494,
-0.5773795247,
0.129460454,
-0.1186997294,
-0.1146923974,
-0.0334540457,
-0.1582231671,
0.1593217105,
0.020614991,
0.3829501867,
0.1888182312,
-0.0159400646,
-0.1086774766,
-0.1195141748,
-0.1931985319,
-0.0825988874,
-0.1219008714,
0.2010165602,
-0.3226899803,
-0.0618356615,
-0.1457770467,
0.038975928,
-0.3498083949,
0.0937143192,
0.1062969789,
-0.0201894138,
0.3603177369,
0.1459479332,
0.7699726224,
0.048815161,
0.1150308847,
-0.0913875476,
-0.353649199,
-0.4042430222,
0.3688688576,
0.3255911171,
0.2565357983,
-0.1063772365,
0.0097641386,
-0.1644826233,
0.0945067257,
-0.04354655,
0.3211485744,
-0.2544759512,
0.2145047933,
-0.0240108576,
0.0171401687,
0.0991312191,
-0.0556259044,
-0.0026464916,
0.3410544395,
-0.2067562938,
-0.6047096252,
0.6198091507,
-0.212931633,
-0.2395552248,
0.1537303925,
0.1545257866,
0.2213794589,
-0.2187473923,
-0.5100530386,
0.0247500949,
0.3773825169,
0.0190039705,
-0.4423446655,
-0.0037064492,
-0.1927573532,
0.051444225,
0.113560237,
0.3062537014,
0.1166288406,
-0.1932859123,
0.1991046965,
-0.2543466091
]
|
https://github.com/huggingface/datasets/issues/1989 | Question/problem with dataset labels | No problem at all. I thought I'd be able to solve this but I'm unable to replicate the issue :/ | Hi, I'm using a dataset with two labels "nurse" and "not nurse". For whatever reason (that I don't understand), I get an error that I think comes from the datasets package (using csv). Everything works fine if the labels are "nurse" and "surgeon".
This is the trace I get:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: not nurse
```
Any ideas how to fix this? For now, I'll probably make them numeric. | 20 | Question/problem with dataset labels
Hi, I'm using a dataset with two labels "nurse" and "not nurse". For whatever reason (that I don't understand), I get an error that I think comes from the datasets package (using csv). Everything works fine if the labels are "nurse" and "surgeon".
This is the trace I get:
```
File "../../../models/tr-4.3.2/run_puppets.py", line 523, in <module>
main()
File "../../../models/tr-4.3.2/run_puppets.py", line 249, in main
datasets = load_dataset("csv", data_files=data_files)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 572, in download_and_prepare
self._download_and_prepare(
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 650, in _download_and_prepare
self._prepare_split(split_generator, **prepare_split_kwargs)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/builder.py", line 1028, in _prepare_split
writer.write_table(table)
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/datasets/arrow_writer.py", line 292, in write_table
pa_table = pa_table.cast(self._schema)
File "pyarrow/table.pxi", line 1311, in pyarrow.lib.Table.cast
File "pyarrow/table.pxi", line 265, in pyarrow.lib.ChunkedArray.cast
File "/dccstor/redrug_ier/envs/last-tr/lib/python3.8/site-packages/pyarrow/compute.py", line 87, in cast
return call_function("cast", [arr], options)
File "pyarrow/_compute.pyx", line 298, in pyarrow._compute.call_function
File "pyarrow/_compute.pyx", line 192, in pyarrow._compute.Function.call
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 84, in pyarrow.lib.check_status
pyarrow.lib.ArrowInvalid: Failed to parse string: not nurse
```
Any ideas how to fix this? For now, I'll probably make them numeric.
No problem at all. I thought I'd be able to solve this but I'm unable to replicate the issue :/ | [
0.1789148301,
-0.0573230609,
0.0051793531,
0.1343671232,
0.4113537371,
0.3502124548,
0.6419945955,
0.1637074798,
-0.1613179296,
0.0723498836,
0.1788543165,
0.0638753474,
-0.0607729964,
0.030525703,
-0.130230993,
-0.1193704456,
0.0890837312,
0.1734791994,
0.1816426963,
-0.04446676,
-0.2867425382,
-0.0398259945,
-0.156679973,
0.3662885129,
-0.297205627,
-0.3766838312,
0.2105430812,
-0.1205282882,
-0.1131331921,
-0.4633954763,
0.2718095183,
0.0861200094,
0.0026800728,
0.4034268558,
-0.0001030963,
0.058756806,
0.2967477739,
0.1559889019,
-0.3984076679,
-0.4321287572,
-0.1893597841,
-0.0234080795,
0.0345638432,
-0.3044767976,
0.0979833305,
-0.2846682072,
-0.0905410498,
-0.2756658196,
0.1046864912,
0.4386059344,
0.3266399205,
0.2373396754,
-0.2065001428,
-0.2293031216,
0.2311526835,
0.1250885725,
-0.0305089504,
0.0494753644,
-0.1344386041,
-0.0064582187,
0.4515704513,
0.460914433,
-0.2723956704,
0.2647709846,
0.1353648007,
0.1463635266,
0.1377247274,
-0.3910481632,
0.2763096094,
0.1961432248,
0.7452604771,
-0.2964998186,
-0.2943911254,
-0.1697053015,
0.1373766065,
-0.4167195559,
0.1813390404,
0.0700832456,
0.0063756779,
-0.0470148437,
-0.0331627578,
0.0610270314,
0.0807417855,
0.0881804153,
-0.0580748878,
0.0383284204,
-0.0986412764,
0.3288168907,
0.1125301495,
-0.0747507066,
0.2975301445,
-0.0059941988,
-0.0885701999,
0.0415449142,
-0.4325241745,
-0.0296084415,
-0.0368810184,
0.0516759381,
-0.1181376055,
-0.038740769,
0.0183869507,
-0.3749563098,
-0.1474356651,
0.3403449953,
0.2151344717,
0.2662764788,
0.2706995606,
0.5616863966,
0.0911723822,
-0.0761627182,
0.0308040548,
-0.0558074787,
-0.0015739995,
-0.4626432061,
0.2433210015,
0.0989312604,
0.2934703231,
-0.2398585975,
-0.5455213189,
0.1924421936,
-0.0281014666,
0.0940956026,
0.1541178226,
0.3049503565,
0.0221220106,
0.2065178901,
0.0793469325,
0.193608433,
-0.0091703041,
-0.1172054783,
-0.2069241703,
0.0976080745,
-0.2002791017,
-0.150106892,
0.0502697304,
-0.0720530748,
0.1124490499,
0.0640597194,
-0.0010049042,
-0.0760566816,
-0.0163034722,
-0.3864641488,
-0.012748206,
0.3588934243,
-0.1032633781,
0.420350641,
0.2520543337,
-0.4185501039,
0.0596993491,
0.1802113652,
-0.3688521683,
-0.0338135473,
-0.2121574432,
0.2862125635,
-0.1030441746,
-0.187981084,
-0.1200913265,
0.0468237363,
0.4193198979,
-0.2145542502,
0.1575898081,
-0.3543840945,
-0.3093222678,
-0.2777702212,
-0.0663038418,
0.2214321792,
-0.6722466946,
0.004389429,
-0.1475467384,
-0.0291129835,
0.0528065376,
0.2960194051,
-0.0810041502,
0.2062916607,
-0.0705919117,
0.0303497873,
0.1395514011,
-0.390691489,
-0.1104637682,
0.1035439447,
-0.1420033723,
-0.2442642748,
0.0704807937,
-0.0403395221,
0.0917313397,
-0.0916260481,
0.0582318865,
-0.1318774968,
-0.1996321678,
-0.0951335654,
-0.1437036842,
0.0538111962,
0.653909564,
0.0927327722,
-0.016800072,
0.0756470934,
-0.1002285406,
-0.3448368311,
-0.0425327159,
-0.1009554341,
0.2062197328,
0.2147901356,
0.0556404777,
0.2983597219,
0.1459521502,
-0.0644702911,
-0.4424965978,
0.1017011777,
-0.0400518253,
0.1395645589,
-0.145143196,
-0.1923438162,
-0.3297647238,
-0.0537859611,
-0.2079762369,
0.0183238313,
0.1633132249,
0.0683607757,
-0.2214221507,
-0.0204832722,
0.0140843056,
-0.075884521,
-0.0172140431,
0.1093305945,
-0.1040251106,
0.2656517625,
-0.0951162279,
0.1168940216,
-0.0267393459,
0.2531200051,
0.4326063693,
0.2400517166,
-0.0466214195,
0.367023617,
0.0848606005,
-0.3270231187,
0.0078404322,
-0.172658667,
0.0181292761,
-0.0838742778,
-0.0163266249,
0.1145247817,
0.2768396139,
-0.1085973084,
-0.0847191364,
0.1411861628,
-0.1772004515,
0.2762841582,
-0.1619747579,
0.2004606873,
0.2599629462,
-0.1093928516,
0.1251222938,
-0.2127112299,
0.2158810794,
-0.1297985166,
0.2343143374,
0.007142223,
-0.2617177367,
0.0677190349,
0.4220539927,
0.0015310015,
-0.0035239446,
0.009774005,
-0.1586676836,
0.1657464355,
-0.0130180242,
0.595718503,
0.352842629,
0.2059556991,
-0.1638759673,
-0.0582683198,
-0.2622588873,
-0.1369839609,
0.2124615908,
0.0951014385,
0.0298523773,
0.0572962686,
0.0261101257,
-0.0664390028,
-0.1765725613,
-0.2433390021,
0.2082311809,
0.3464297354,
-0.51031214,
-0.0029065625,
-0.3452727795,
-0.2318637222,
-0.3185971677,
-0.0987910777,
-0.2351591289,
-0.4590440094,
0.0904379636,
-0.0774915814,
-0.1903930455,
0.1450763792,
-0.0375638828,
0.0079173651,
-0.0910730362,
0.1520518214,
-0.0975839868,
-0.4702498615,
-0.2045714557,
0.1811123341,
0.0074800067,
0.0469533242,
0.4813601077,
-0.1431986392,
-0.1245555803,
-0.0785720423,
-0.2953169942,
0.0040264316,
-0.2730659246,
0.1366041899,
0.1816231608,
0.103859432,
0.0084615927,
-0.1486845016,
0.2930162251,
-0.0715196803,
0.0246398468,
0.1069570333,
0.0452330634,
-0.2920732796,
-0.2664697468,
-0.4750192463,
-0.4745301306,
-0.1774818748,
0.0669479594,
-0.075908266,
-0.0335285738,
0.5198025703,
0.0684420913,
0.0656812266,
0.0931449234,
0.023252707,
-0.3467434645,
-0.0935407355,
0.2490479201,
-0.179091692,
-0.210245356,
0.1960575432,
0.1093095243,
0.1175282896,
-0.20393309,
-0.3139223158,
0.0728625953,
-0.0576012135,
0.058944717,
0.0813103691,
0.1948962212,
0.2138828039,
0.2671021223,
-0.1480035037,
-0.3270511925,
-0.3317118883,
0.1381734908,
-0.0719676912,
0.1306350976,
-0.1062558591,
0.294085592,
-0.152083829,
0.326508671,
0.0217723008,
-0.2126558274,
0.191888988,
-0.0478476658,
0.5207321048,
0.068380706,
-0.4193751216,
0.0658852011,
-0.1191071793,
0.0269460715,
0.1760428697,
-0.3196389079,
0.0379566401,
-0.1479681134,
0.3946042061,
-0.2478049397,
-0.0996567011,
-0.1006427184,
-0.1501683444,
0.0405269749,
0.1169003174,
-0.0409751162,
-0.2084082365,
-0.1915850341,
-0.2512801886,
0.0578238554,
-0.0954614133,
-0.087551482,
-0.533575058,
-0.2334993929,
-0.1649958491,
0.2940982878,
0.1574513167,
0.3346639276,
-0.1785171777,
0.2454715222,
0.0477584898,
0.2458998859,
0.6149811745,
-0.4832390547,
0.154702425,
0.2263719141,
0.3931272328,
-0.1780015528,
-0.1272553653,
-0.1692574769,
0.2947262228,
-0.2957772911,
0.0259887744,
-0.1555344164,
-0.0910770446,
0.2631006241,
0.1554057747,
-0.1223803461,
-0.3149805069,
-0.2309516966,
-0.1793929189,
-0.1272854507,
-0.0533969514,
0.0635773465,
0.1866380721,
-0.3784870207,
0.0986478776,
0.1408236176,
0.0735073909,
0.2715045512,
0.1186348274,
0.2232429981,
0.0302767064,
0.197361365,
0.317402184,
0.2587257028,
0.5099822879,
0.5483981967,
-0.3618466854,
-0.5113294125,
-0.1194789112,
-0.1147691086,
0.2007077336,
0.0650010705,
-0.1422151178,
0.1660957336,
-0.0506398827,
0.185458824,
0.0961978808,
0.0223078672,
0.4922952354,
0.1605764627,
-0.4898479283,
-0.5388931632,
0.2713592649,
0.0392414108,
-0.0478406474,
0.1281552166,
0.0797321871,
-0.3407573402,
0.2059525549,
-0.1559936553,
0.6908729672,
0.0837977454,
-0.0510304905,
-0.0170310941,
-0.2261731625,
0.7132234573,
-0.1187111512,
0.0007714578,
-0.3614293039,
-0.3074660599,
-0.0622299947,
-0.0221186746,
0.0234751571,
0.4506933987,
-0.3085790277,
0.3788379431,
-0.3285133839,
0.2046867758,
0.028750021,
-0.0740614757,
0.2494399399,
-0.1323137879,
-0.0824863017,
0.2010339946,
-0.1576190293,
-0.1343754977,
-0.1674345434,
0.0419054106,
-0.2318485528,
-0.2680606246,
-0.2262603641,
0.08226078,
-0.2538248897,
0.1415783167,
-0.0856540874,
-0.0167195015,
0.2168119252,
0.2025102079,
0.4863218665,
0.0789240599,
-0.1482862979,
0.1838956922,
0.0421487242,
0.1370235533,
0.0343489051,
0.0537468866,
0.4150622785,
0.1160496846,
-0.2699809074,
0.1285305768,
-0.0795674771,
0.1759498864,
-0.0870310813,
0.0838943273,
-0.0983974338,
-0.3653748333,
0.0326472521,
-0.0770386755,
0.2287106365,
-0.2537733614,
0.1773460358,
0.0519831851,
-0.0466800705,
0.2120788842,
-0.2636915445,
-0.1465975195,
-0.0457466282,
0.1892329752,
0.0259641036,
0.1933565587,
0.2472447455,
0.0259730872,
-0.3513919413,
-0.2565112412,
0.038952101,
0.0239994247,
-0.6778352261,
0.2459824234,
0.2533547282,
0.0759458542,
-0.057189174,
0.2232685387,
-0.0158301294,
0.0710849985,
0.0610140152,
-0.5274376869,
-0.2186500877,
-0.00467043,
-0.1847544909,
0.1169297621,
0.2478654385,
0.4110050797,
0.1337576956,
0.0714501962,
-0.3641543388,
-0.020954119,
-0.172394067,
0.2980129123,
0.1525106579,
0.0199611541,
0.2820312381,
-0.1788062751,
0.218216911,
0.0007437372,
0.007750046,
-0.2498184741,
-0.1872092783,
0.1124106348,
0.1352093369,
-0.1972157359,
-0.0634114593,
-0.1643812805,
0.0578369349,
-0.0719840601,
-0.0172511172,
0.3105739653,
0.0032078198,
0.3735309839,
-0.1509219557,
0.2327846885,
-0.0463298075,
0.2182779461,
0.0127172945,
-0.114001587,
0.0742451549,
0.1729656607,
-0.1127646267,
0.0194780901,
-0.1750794947,
0.0847947747,
-0.1170051619,
-0.11792963,
0.1342932731,
-0.568980515,
0.2959564924,
0.193638131,
0.449362725,
0.2781369686,
-0.1868256927,
-0.0707662776,
0.3826093972,
0.307926774,
-0.3656698763,
-0.1683599651,
0.1630016863,
0.1655610651,
0.0003906599,
0.0756075159,
0.060725417,
-0.0310598556,
0.0448926538,
0.0080858618,
0.4554387927,
-0.4376546144,
0.2349053174,
0.5269825459,
-0.0619708523,
0.0999840051,
0.4967942238,
0.1731765717,
0.0635329187,
0.5881978869,
0.0346502028,
0.1773207486,
0.1874702275,
-0.1379599422,
-0.0754204541,
-0.172217235,
0.1809182614,
0.0969726592,
0.0188562367,
-0.0428314172,
-0.0954477713,
0.3011343777,
-0.3839455247,
0.0041103126,
-0.0057690036,
0.1111971214,
-0.2255034149,
-0.1060157418,
-0.1562631726,
-0.0987095013,
-0.1664459705,
-0.1973160356,
0.0140280882,
-0.1700504124,
0.3556354642,
-0.0742809027,
-0.1959641278,
-0.4565812349,
-0.186778456,
0.305419594,
0.065081425,
-0.2473097742,
0.0163199063,
0.2487559468,
-0.1455008835,
0.3213778436,
0.086239703,
0.6039388776,
0.1713752747,
0.0897912681,
-0.3410916328,
-0.0972951949,
-0.088580206,
0.1516636312,
0.215568006,
0.0459204242,
-0.0368339717,
0.3922656178,
0.2018781453,
-0.1707493216,
0.3085803986,
0.3357422054,
0.2227042168,
-0.170619756,
-0.0630670115,
-0.1339656562,
-0.2573547959,
-0.3266397119,
0.1013188735,
-0.2697949111,
0.1159574091,
0.4622558057,
-0.0415135659,
0.0840901211,
-0.2942293584,
0.1462800503,
0.1317104101,
0.3118901849,
0.2739442885,
-0.0476244502,
-0.3456508517,
0.1615712494,
-0.5773795247,
0.129460454,
-0.1186997294,
-0.1146923974,
-0.0334540457,
-0.1582231671,
0.1593217105,
0.020614991,
0.3829501867,
0.1888182312,
-0.0159400646,
-0.1086774766,
-0.1195141748,
-0.1931985319,
-0.0825988874,
-0.1219008714,
0.2010165602,
-0.3226899803,
-0.0618356615,
-0.1457770467,
0.038975928,
-0.3498083949,
0.0937143192,
0.1062969789,
-0.0201894138,
0.3603177369,
0.1459479332,
0.7699726224,
0.048815161,
0.1150308847,
-0.0913875476,
-0.353649199,
-0.4042430222,
0.3688688576,
0.3255911171,
0.2565357983,
-0.1063772365,
0.0097641386,
-0.1644826233,
0.0945067257,
-0.04354655,
0.3211485744,
-0.2544759512,
0.2145047933,
-0.0240108576,
0.0171401687,
0.0991312191,
-0.0556259044,
-0.0026464916,
0.3410544395,
-0.2067562938,
-0.6047096252,
0.6198091507,
-0.212931633,
-0.2395552248,
0.1537303925,
0.1545257866,
0.2213794589,
-0.2187473923,
-0.5100530386,
0.0247500949,
0.3773825169,
0.0190039705,
-0.4423446655,
-0.0037064492,
-0.1927573532,
0.051444225,
0.113560237,
0.3062537014,
0.1166288406,
-0.1932859123,
0.1991046965,
-0.2543466091
]
|
https://github.com/huggingface/datasets/issues/1988 | Readme.md is misleading about kinds of datasets? | Hi ! Yes it's possible to use image data. There are already a few of them available (MNIST, CIFAR..) | Hi!
At the README.MD, you say: "efficient data pre-processing: simple, fast and reproducible data pre-processing for the above public datasets as well as your own local datasets in CSV/JSON/text. "
But here:
https://github.com/huggingface/datasets/blob/master/templates/new_dataset_script.py#L82-L117
You mention other kinds of datasets, with images and so on. I'm confused.
Is it possible to use it to store, say, imagenet locally? | 19 | Readme.md is misleading about kinds of datasets?
Hi!
At the README.MD, you say: "efficient data pre-processing: simple, fast and reproducible data pre-processing for the above public datasets as well as your own local datasets in CSV/JSON/text. "
But here:
https://github.com/huggingface/datasets/blob/master/templates/new_dataset_script.py#L82-L117
You mention other kinds of datasets, with images and so on. I'm confused.
Is it possible to use it to store, say, imagenet locally?
Hi ! Yes it's possible to use image data. There are already a few of them available (MNIST, CIFAR..) | [
-0.10489434,
-0.407784611,
-0.1252108216,
0.3528690636,
0.2064329088,
0.0773041323,
0.2821690142,
-0.0132196387,
0.1014457271,
-0.092151098,
-0.2971546054,
-0.0767040029,
-0.0591902919,
0.541465342,
0.5551631451,
-0.0550171807,
0.1947558224,
0.0077368575,
-0.1606639922,
0.0243541952,
-0.1311548799,
-0.0184783395,
-0.1919616163,
-0.0560768396,
-0.2441981137,
0.0734434947,
-0.2431033105,
0.2023607194,
-0.352455616,
-0.3153181374,
0.1265479028,
0.142026037,
0.2038790584,
0.1515561938,
-0.000114474,
0.0261505768,
0.2794186175,
-0.2648742795,
-0.0799025968,
-0.2754316926,
-0.2100360543,
-0.1958542168,
0.1365065277,
-0.3097443879,
-0.0344097987,
-0.3639691174,
0.3208797574,
-0.202970013,
0.4163269997,
0.3321008682,
0.1734584868,
0.1770460904,
-0.0120294159,
0.0715580881,
0.199496448,
0.7043313384,
-0.2353238761,
0.0765625387,
0.3966985643,
0.2751346529,
0.1317596138,
0.1904015094,
-0.1471002102,
0.2446240336,
0.6670562625,
0.284781754,
-0.0634087771,
-0.5953474641,
0.1768102944,
0.3605560958,
0.7247246504,
-0.2514559329,
-0.3772625923,
-0.2556188405,
-0.3492875397,
-0.1461131275,
-0.0531080402,
0.2958315015,
-0.0094538759,
0.234073922,
-0.5905613303,
-0.275891006,
-0.1851314157,
-0.0329568088,
0.0618031025,
0.2776290178,
-0.3768873215,
0.2667974532,
0.1519193798,
0.1478043944,
0.0193915926,
-0.2411189824,
0.090468429,
0.213638857,
0.1061237454,
-0.2127498537,
-0.3010907769,
0.1735036969,
0.4054277837,
0.0513449758,
-0.0248879585,
-0.0001729788,
-0.2388936728,
0.263392657,
0.3330247998,
-0.0660581142,
-0.0647344738,
-0.0764097646,
0.2803306282,
-0.0680023134,
0.287571162,
-0.0922327489,
-0.2132638246,
-0.0572033785,
-0.1317409575,
-0.3184928,
0.1354008168,
-0.2608079016,
-0.0464894921,
0.0581914447,
0.1385325938,
-0.1853194237,
-0.1996646672,
0.23537907,
-0.0096433898,
-0.1611841917,
0.1309795082,
0.2234883308,
0.1141235158,
-0.1701413095,
0.008983653,
0.1949694604,
-0.2847525477,
0.1963900477,
0.0610170849,
-0.2041507661,
0.3392303288,
-0.1049998701,
0.2044094503,
0.0342723578,
0.1778148562,
-0.2119625509,
0.3175320625,
0.4655560851,
-0.091653347,
0.0578257143,
0.079101257,
0.0507050641,
-0.4007252753,
0.1867388487,
-0.4734028578,
-0.2855851054,
-0.1491547823,
0.036601577,
-0.1271824837,
0.0141972387,
-0.4138628542,
0.2678914368,
0.1148288399,
0.1325328797,
0.2375144511,
0.0565747432,
-0.3937696218,
-0.2800177932,
0.2161932737,
0.2000335753,
-0.4700540304,
0.1166010052,
-0.0538983569,
-0.2484966069,
-0.0362887904,
0.2947600186,
-0.1932139695,
0.1562078446,
-0.2128871083,
0.1740452349,
0.1366380602,
-0.4921629727,
0.2029120922,
0.3513149023,
0.3671302795,
-0.1108641177,
0.0441716202,
0.1537507027,
0.042913314,
0.0106787477,
-0.2065613419,
0.2118646652,
0.0240101311,
-0.1647772491,
-0.0510588028,
-0.4474026561,
-0.0594861805,
0.2002525628,
0.09965197,
0.1039629802,
0.1326236427,
-0.0051944219,
0.0929167867,
-0.0749802962,
0.3068464398,
0.0156941302,
0.1508459002,
0.1359283924,
0.1380653977,
-0.0318660401,
-0.4235832095,
0.1685134023,
0.063308686,
0.0763664171,
0.0761730149,
-0.3972410858,
-0.0179491863,
-0.096662581,
-0.0226481538,
-0.3566536009,
0.0199157819,
-0.0699633732,
0.1150774062,
-0.1959698349,
-0.5987905264,
0.3285927176,
-0.1511592269,
0.0230141971,
-0.4239827693,
0.1418730766,
-0.1346036345,
0.1803492159,
-0.0286203828,
-0.0403108485,
-0.2251121104,
-0.3223880529,
0.2558921576,
0.3308747709,
0.0934398323,
0.0722601563,
0.1576123387,
0.5650747418,
0.1312619597,
-0.2301092744,
0.3880788684,
-0.1910668612,
0.2906060815,
-0.0601734631,
-0.4578298032,
0.4475468397,
-0.0550349839,
0.1308478266,
0.0638445243,
-0.1445368379,
0.2165029198,
-0.0154156238,
-0.2617866099,
-0.1515649259,
-0.0750199258,
0.0945292786,
0.3611968756,
-0.0716758147,
-0.3708128333,
0.0365553945,
0.2601163685,
-0.1820849776,
-0.0158426426,
0.2417080998,
-0.115055576,
-0.3044472039,
0.1658322662,
0.0687475502,
0.4986748099,
0.039202638,
0.0669746622,
0.2871891558,
0.0804617256,
-0.1671083272,
0.1338183135,
0.0213153828,
0.2222682387,
-0.0037231592,
-0.2135403305,
-0.0318362974,
-0.1227990091,
0.0990000591,
0.0228220671,
0.0743143484,
-0.2289335728,
0.2501445711,
-0.1536979526,
-0.2292582691,
0.0220877361,
0.0896798745,
-0.3034617305,
0.0047840364,
-0.1613830924,
-0.0531566143,
0.0799913481,
-0.1376591176,
-0.0357056782,
0.4570160806,
-0.1464420557,
0.1405965984,
-0.3284786046,
0.071608983,
0.0750910938,
0.0750454292,
0.3216887712,
0.1382005513,
0.4002685845,
-0.3740537465,
-0.1360501051,
-0.3646130264,
-0.1546107382,
0.4024239779,
-0.2423147261,
0.3959169686,
0.3424026966,
0.2233856916,
-0.1198297143,
0.2312307209,
0.1523536146,
-0.2863829732,
-0.1678372025,
-0.3098676205,
-0.0997557491,
-0.2488843948,
-0.311501056,
-0.0051126624,
-0.3916943967,
-0.1449798942,
0.2766245008,
0.3025511503,
0.3917852044,
0.2861987948,
0.1617549807,
0.3849446476,
-0.2790232003,
0.0002964958,
-0.3693481386,
-0.6604459286,
0.2806851864,
-0.2496241778,
-0.3559563756,
0.2686174214,
0.1082689017,
0.1110764667,
0.052417513,
-0.5647810698,
-0.3803994954,
0.073514156,
-0.0298806075,
0.317315042,
0.2644762099,
0.141149655,
-0.3616520464,
0.021610586,
-0.2539396286,
-0.2714327574,
-0.0720844716,
-0.0069527207,
0.1494465619,
0.3848906755,
0.2619083226,
-0.0657448396,
0.4962345362,
0.1023641825,
-0.006929446,
0.4028249681,
0.0429874323,
0.4390584528,
-0.5036190152,
0.1889147609,
0.3229768276,
-0.2280292958,
-0.0893527195,
0.2797308266,
0.1519522518,
-0.0002821078,
-0.2032934427,
0.0741906241,
-0.2951330245,
0.086004585,
0.1225503013,
0.3008069396,
0.0357660577,
-0.0828718245,
-0.211348787,
0.0658425912,
0.0793487206,
-0.1980215013,
0.5932249427,
0.3951218724,
0.0423818156,
-0.4509611726,
-0.0493150875,
-0.4759035707,
0.2078606337,
-0.2291044444,
0.0366467312,
-0.06227649,
0.171050787,
0.35136199,
-0.2008669525,
0.3242229223,
-0.4471011162,
-0.3030696213,
0.1148628294,
-0.2994035482,
-0.1905005872,
0.0899869576,
-0.1753986627,
0.1934818923,
-0.0422459133,
0.4469902813,
-0.2695122361,
-0.330999881,
0.215667069,
0.0433718078,
-0.2091080993,
-0.1733766347,
-0.0237394739,
-0.3795312643,
-0.4606887996,
-0.3016346991,
0.1094655469,
-0.3531166613,
0.0789416954,
0.1045582891,
0.0741393492,
0.2746050358,
0.0787392184,
0.0344859958,
0.0890975371,
-0.0308660679,
-0.1190999225,
0.2502966523,
0.2833505273,
0.1083385646,
0.6675298214,
-0.2094114125,
-0.1703837812,
0.166661039,
-0.0685448721,
0.3022338748,
0.2958959937,
-0.0461921468,
-0.0509173237,
0.2474733442,
-0.0380420052,
-0.5824604034,
0.0347115472,
0.2892960906,
-0.1367737651,
-0.4954518676,
-0.5957934856,
0.6540588737,
0.0735012889,
-0.0504068397,
0.0816586539,
0.215545103,
-0.3724493384,
0.1935535222,
0.543510735,
1.0711700916,
-0.2064468265,
0.4783606231,
-0.0353164971,
-0.0264179669,
0.3445595503,
-0.1600822657,
-0.2060886174,
-0.2130373567,
0.0525087789,
-0.2116366625,
-0.0434282795,
0.2980109155,
0.074361451,
-0.2512976229,
0.0711922422,
0.0467815064,
0.1992853135,
-0.2622815669,
0.3801472783,
-0.0763471425,
-0.2325715572,
-0.0787088349,
0.1511822343,
0.1660846621,
0.0884915367,
-0.088343516,
0.0518041775,
-0.1285293102,
-0.0104413014,
-0.2773343325,
-0.4066329896,
0.0938110426,
-0.212422967,
0.0439091437,
-0.0421204641,
0.1966651827,
0.0610067807,
0.4875641167,
-0.0119993109,
-0.5061596632,
-0.0909201056,
-0.1689058393,
-0.1601187885,
0.001537518,
-0.3381933272,
0.2413758934,
-0.1594565362,
0.0756151676,
-0.0897732005,
-0.0050942241,
-0.2896894813,
-0.115263626,
0.0730125532,
0.0224783439,
-0.1160960346,
-0.3256320953,
0.0479333997,
-0.1418658048,
0.0119073689,
0.071586594,
0.2889599204,
0.0282930117,
0.2428250313,
-0.0221461952,
-0.1877586991,
-0.1326959729,
0.0051655411,
0.1976089031,
-0.021600632,
-0.0415341109,
0.115525946,
-0.2569546998,
-0.1879383773,
0.2555363774,
0.3657173514,
-0.2913582027,
0.0343417116,
0.2049905211,
-0.038592644,
-0.2323975116,
0.3837563396,
0.2756187618,
-0.25395298,
-0.1566253901,
-0.4555264711,
-0.4556473196,
0.2915143967,
0.055008918,
0.272264272,
0.0974756107,
-0.1319727004,
0.3784187436,
0.1438316107,
-0.2696528137,
0.2071359456,
0.194393158,
0.2276331037,
0.3067965209,
0.1615452021,
0.1273258477,
-0.1219339296,
-0.1021168381,
0.000531199,
-0.5738063455,
-0.1093949378,
-0.082992278,
0.2434397638,
0.2102244496,
-0.6044507623,
0.2512055039,
-0.1884343773,
-0.4141261876,
-0.1705607176,
0.056787774,
0.3243684471,
-0.1336842179,
-0.1698005199,
0.3111150861,
0.03588631,
0.1018795595,
0.0126811462,
0.1406434178,
0.2875846624,
0.1090642884,
0.005835609,
-0.1595470309,
-0.0762466714,
0.2651562095,
0.224155128,
0.3071345091,
0.0347260609,
0.450304836,
-0.0871045515,
0.2993297577,
-0.099581711,
0.6628456116,
0.4894656241,
-0.2899218202,
0.2158206254,
0.339931041,
0.0476659462,
-0.1515320987,
-0.0237077586,
0.1133869365,
0.1913728863,
-0.1148005798,
0.3257424235,
0.5763187408,
0.3541562259,
-0.0552238151,
-0.0920712575,
0.4977540076,
-0.2361808717,
-0.0451125391,
-0.0041513019,
-0.2009322643,
0.2144484073,
0.3568069935,
0.2959001958,
0.2447047681,
0.394937396,
-0.0449643508,
0.3169945478,
0.2117175013,
0.3111314774,
0.1836907715,
-0.1737134457,
0.341905266,
0.35210976,
-0.0029114631,
0.2933191061,
0.0115052955,
0.2548394501,
-0.0377793014,
0.0649411157,
-0.2181035578,
0.2974997461,
0.0736963376,
-0.2234392613,
-0.1065744683,
-0.2485698313,
0.0351707563,
0.0781804249,
-0.2575294673,
-0.4391989708,
0.4528003335,
-0.1613835692,
0.0814208835,
-0.0117286323,
0.2299821526,
-0.1840505153,
-0.0126002338,
-0.0147154238,
0.4757889509,
-0.0388569087,
0.1751499176,
0.2862820327,
0.4984342456,
0.4124893546,
0.295129925,
0.0415234752,
0.1162274554,
-0.2170935571,
-0.0240295641,
-0.1073996201,
0.295620203,
0.105256632,
-0.2680222988,
0.3524371386,
0.0467499904,
-0.0150580965,
0.1611463428,
-0.2480763197,
-0.094732061,
-0.257894218,
-0.0653259754,
-0.0398038253,
-0.0521666333,
-0.019610228,
0.0834796727,
-0.4652505815,
-0.1512022763,
0.4083548486,
-0.1822609901,
0.2170267999,
-0.0191246085,
0.0429894328,
-0.1277042627,
0.5674462318,
0.1758660525,
0.2255424708,
-0.1872520447,
-0.0654118434,
-0.6191355586,
0.0173135493,
-0.1485693604,
-0.0276774447,
-0.0342555717,
0.1928009242,
0.1183424965,
0.3820802867,
0.1264027953,
-0.1649759412,
0.078174524,
0.1624614894,
0.0699720532,
0.0281058177,
-0.1390953958,
0.538762629,
-0.1229069084,
-0.0895444676,
0.3787789941,
-0.0359523036,
-0.1172062829,
-0.2300397456,
0.2673722208,
0.1256122589,
0.0506163873,
0.4510185421,
0.0111476192,
0.104297258,
-0.2395533025,
-0.0749336109,
-0.2782075107,
0.0913220048,
-0.3062449992,
0.1464632004,
0.0392668769,
-0.0720212013,
-0.5130120516,
-0.1260092109,
-0.1060917899,
-0.0476450026,
-0.2147376835,
-0.1384328157,
-0.2805689573,
-0.0222003385,
-0.3666783273,
-0.1302179694,
0.079945311,
0.191241473,
0.0207772497,
-0.3268557191,
-0.4230949581,
-0.0962444916,
0.573299408,
-0.0807914287,
-0.094352603,
0.0504301339,
-0.2250855267,
0.0867059678,
-0.1694976687,
-0.8003808856,
-0.024474632,
0.2794878483,
0.1144611835,
-0.0494966209,
0.1213409677,
0.0376006551,
-0.1471038163,
-0.3729901314,
0.352612257,
-0.0036820259,
-0.1638883948,
-0.3388902545,
-0.4334890246
]
|
https://github.com/huggingface/datasets/issues/1983 | The size of CoNLL-2003 is not consistant with the official release. | Hi,
if you inspect the raw data, you can find there are 946 occurrences of `-DOCSTART- -X- -X- O` in the train split and `14041 + 946 = 14987`, which is exactly the number of sentences the authors report. `-DOCSTART-` is a special line that acts as a boundary between two different documents and is filtered out in our implementation.
@lhoestq What do you think about including these lines? ([Link](https://github.com/flairNLP/flair/issues/1097) to a similar issue in the flairNLP repo) | Thanks for the dataset sharing! But when I use conll-2003, I meet some questions.
The statistics of conll-2003 in this repo is :
\#train 14041 \#dev 3250 \#test 3453
While the official statistics is:
\#train 14987 \#dev 3466 \#test 3684
Wish for your reply~ | 78 | The size of CoNLL-2003 is not consistant with the official release.
Thanks for the dataset sharing! But when I use conll-2003, I meet some questions.
The statistics of conll-2003 in this repo is :
\#train 14041 \#dev 3250 \#test 3453
While the official statistics is:
\#train 14987 \#dev 3466 \#test 3684
Wish for your reply~
Hi,
if you inspect the raw data, you can find there are 946 occurrences of `-DOCSTART- -X- -X- O` in the train split and `14041 + 946 = 14987`, which is exactly the number of sentences the authors report. `-DOCSTART-` is a special line that acts as a boundary between two different documents and is filtered out in our implementation.
@lhoestq What do you think about including these lines? ([Link](https://github.com/flairNLP/flair/issues/1097) to a similar issue in the flairNLP repo) | [
0.1660704464,
-0.3441514969,
-0.0265199859,
0.371819526,
-0.3747007549,
-0.0020878583,
0.0613713749,
-0.0708982125,
-0.9394968748,
-0.0062832027,
0.1252354681,
0.1568081975,
0.0813579783,
0.0008894681,
-0.0184588209,
0.0292957257,
0.1648516804,
-0.0400792062,
0.2795079052,
-0.1671277732,
-0.0582849495,
0.4750360548,
-0.5303708911,
0.0231518485,
-0.8211599588,
0.0690075532,
-0.1038990021,
0.164584592,
-0.3679313362,
-0.3551765084,
0.2766614556,
0.0723338798,
0.1248625219,
0.1931931823,
-0.0001210675,
-0.4359930158,
0.2224059403,
-0.0981822535,
-0.1973603517,
0.2145420015,
-0.1930088252,
-0.429505527,
-0.038219545,
-0.1875086129,
0.2144866437,
0.4310619235,
-0.5452499986,
0.2614451051,
0.3766058981,
0.3219398558,
0.1062864885,
-0.0599872246,
0.1251413226,
-0.0609836392,
0.5427100658,
0.0443484299,
-0.0532877371,
0.2690054774,
0.1820477992,
0.2210755348,
-0.0648549572,
0.3887672424,
0.0820058435,
-0.2496803254,
-0.0430985428,
-0.028093826,
-0.2199693322,
-0.2218951732,
0.0012414827,
0.4098291099,
0.4609610736,
-0.2167002112,
-0.3023410439,
-0.6893438101,
0.2730963528,
-0.2258213758,
0.1093589887,
-0.0073540825,
-0.0039546709,
-0.0349639617,
-0.1026144102,
-0.3313957155,
-0.2891083956,
-0.1153046712,
-0.2888514698,
0.3619832695,
-0.0982110798,
0.0420259126,
0.1299822032,
0.2171080261,
0.6810008287,
-0.0352977701,
-0.0374365449,
-0.059974324,
0.0213083252,
-0.282697171,
0.0769813806,
-0.0876313746,
0.4273088574,
-0.3164519668,
-0.3112625778,
-0.4441927969,
0.0422772653,
-0.0517054982,
0.3466987014,
0.0796583295,
0.1834948957,
0.4299294055,
0.0845108852,
-0.0441894047,
0.1412352473,
0.1142534167,
-0.3085032403,
-0.1048448011,
-0.4565194845,
0.0863844007,
-0.4163791537,
-0.413069278,
-0.1330948174,
0.1724146307,
-0.111301817,
-0.2724637687,
0.0154789612,
0.0970013961,
0.2453028113,
0.5682410598,
-0.0069986917,
-0.1539082676,
-0.0962360948,
-0.2754597664,
-0.0487610884,
-0.1259210259,
-0.0837790295,
-0.0407453738,
-0.043136362,
-0.4689646959,
0.1422902942,
-0.0367283635,
0.3424780965,
0.1044099554,
0.0005000352,
-0.1908175796,
0.1123937145,
-0.0760020539,
-0.2280943096,
-0.043192856,
0.0182656888,
0.4265727103,
-0.2598367929,
0.1983310431,
-0.0825975686,
-0.3248426616,
-0.26385957,
0.022616053,
-0.2561663091,
-0.1643794328,
0.619392097,
0.0523145609,
0.2874728143,
-0.1028845981,
0.2342781872,
-0.218329221,
-0.1448467225,
-0.0757277608,
0.2444782555,
0.1550736725,
-0.2432812154,
-0.0339366272,
0.0535975583,
-0.0178702399,
0.0158102419,
0.2335741818,
-0.1454825848,
0.481439501,
-0.099748157,
-0.1582868248,
0.3144388795,
-0.5198950171,
-0.1304041296,
0.1959634572,
0.1512755156,
-0.0103272153,
-0.1246012226,
-0.2870711386,
0.5772901177,
-0.004811198,
0.0324354954,
0.036722295,
0.0223801415,
0.0059986478,
-0.3243201971,
-0.1682893336,
-0.461506933,
-0.3119508922,
-0.0392333455,
-0.4152747989,
-0.3570276499,
-0.1325615346,
0.420612067,
0.1739865392,
-0.071380429,
0.1050240546,
0.2062050253,
0.2779873908,
0.1916782409,
0.3133232594,
-0.1001001596,
-0.019403182,
0.3702383041,
0.1440895498,
0.5833432674,
-0.2024103701,
-0.3424494565,
0.018099023,
0.3618108332,
-0.2660243809,
0.0464324765,
0.0784568787,
0.2490748465,
0.104573831,
-0.2230345309,
0.2294341028,
-0.0686064661,
0.082958959,
-0.0228834748,
-0.1679255068,
0.0076260492,
-0.0514645614,
0.0765400082,
0.3759820759,
0.0501766168,
0.2213460356,
-0.0430643745,
0.1560602635,
0.3733200431,
0.0259111729,
0.159376502,
-0.1503408402,
0.1712959111,
0.0713370442,
0.0039654258,
-0.3762100041,
-0.0671596602,
0.1555730402,
-0.1190157011,
0.2018065751,
0.3937218785,
-0.0849650875,
0.1251068711,
-0.1524042785,
-0.1032811776,
-0.050498683,
-0.0435633734,
-0.4440816641,
-0.0775447041,
0.2097180933,
0.0434643105,
0.1147811562,
-0.1278451383,
0.0794450864,
0.2715922892,
-0.0406669639,
-0.3521394432,
0.3497366011,
-0.3610710502,
-0.0431851968,
0.1686316878,
0.1574980021,
0.1628735363,
0.2707739472,
0.4084943235,
0.0136155896,
-0.1261949688,
-0.2134841084,
0.2715884447,
0.2755282223,
0.269862175,
0.3130464852,
0.1287536323,
0.2043979019,
-0.0576746762,
-0.0522323884,
0.0049609058,
-0.1004542783,
-0.2025469691,
-0.1476151794,
0.0883112773,
-0.3175345063,
-0.0191394947,
-0.264344424,
0.1351877749,
-0.2632876635,
0.2045492977,
-0.2654727101,
-0.1398560554,
-0.0968473703,
-0.4157765508,
0.3907614648,
-0.2395301014,
0.278411895,
0.0863357559,
-0.2383732051,
-0.4046698511,
0.0646501034,
-0.1899422556,
-0.116866976,
0.3373415768,
-0.3670263588,
0.1029951796,
0.14085567,
-0.3336955607,
0.4816082418,
-0.1656109691,
-0.1645953059,
0.2019175738,
-0.3982417881,
-0.088389799,
0.1549082696,
-0.1883399636,
-0.0865185037,
-0.0104287975,
-0.2035204917,
-0.1064207703,
0.1076327488,
-0.1134158,
-0.2887967527,
0.0965063348,
-0.2495455295,
0.0297884997,
-0.1858460605,
0.0634326562,
-0.3321937323,
-0.0946240798,
0.1867457926,
-0.3426501155,
0.2871344686,
-0.1742215902,
-0.3361063302,
0.0613681674,
-0.1169491634,
-0.1123993918,
0.0519327298,
-0.1871530563,
-0.1176810712,
-0.2642967403,
-0.5564438105,
0.3457522988,
-0.0687348098,
0.1330460161,
-0.2591457367,
0.1160944104,
0.3091047704,
0.0254709013,
0.0253793206,
-0.3204878271,
0.0329139344,
-0.0702276006,
-0.0610332601,
0.5930925608,
-0.2280662507,
0.0729789585,
0.1565074772,
0.1225827932,
0.4253534675,
0.0593687259,
-0.048137188,
0.0182670355,
0.164416194,
-0.1071256921,
0.1683373153,
0.2077213824,
-0.0976901948,
-0.1563351303,
0.470561862,
0.364620775,
-0.0054981825,
-0.1388782114,
0.1833248585,
-0.029336853,
-0.2661375403,
0.1739903688,
0.2995580733,
0.3880096674,
-0.1546842754,
0.1660910845,
-0.151252687,
0.0750182942,
-0.2321186662,
-0.1701997966,
0.3782020807,
-0.2222848684,
0.3915689886,
0.386341691,
-0.3913908005,
0.2822631598,
0.329131037,
-0.0832037702,
0.0201571546,
-0.2512382269,
0.0599987246,
0.1080632582,
0.3921407461,
-0.2249245644,
-0.4162835479,
0.4402131438,
0.1864141673,
-0.3669566512,
-0.00298568,
-0.4216748774,
-0.6974323392,
1.1738077402,
0.257347405,
-0.1538089812,
0.0064692805,
0.3078438044,
0.0786393657,
0.0139557831,
-0.2059750259,
-0.1701978743,
-0.1316945255,
-0.2217648774,
0.0581701323,
0.0521630906,
-0.0452027209,
-0.2391822338,
-0.1456110775,
0.4471738636,
-0.1733317077,
-0.0507848561,
0.3019067943,
0.2329543531,
0.0359913222,
0.1739750206,
0.1901965141,
0.2399753034,
0.2120047659,
0.2777104378,
-0.0766570792,
-0.4299618006,
0.163870886,
-0.201599434,
0.5481740832,
0.4593530595,
0.0066288281,
-0.0979799628,
-0.52911973,
-0.0279729255,
0.3044690192,
0.1693530381,
0.2401069254,
-0.0530309454,
-0.3426637053,
-0.0914304331,
0.1091332957,
0.1910517812,
-0.3062945306,
-0.0053375838,
-0.2550164759,
-0.0913891494,
0.0554534011,
0.2086657882,
0.9420134425,
0.2601418197,
0.1926537007,
0.1376912743,
-0.4085134864,
0.6010937095,
0.0820223913,
0.1172552854,
-0.268361181,
0.1279534847,
0.0676626861,
-0.1992435604,
-0.1237356067,
-0.2735462189,
-0.298332274,
-0.1589802355,
-0.3140904307,
-0.172613427,
-0.1010141671,
0.3583070934,
-0.1642760038,
-0.0845817924,
0.4812366962,
0.0399216898,
0.3590168059,
0.0060376981,
-0.1238967255,
0.0394352116,
-0.3739418387,
-0.0988586843,
-0.5869010687,
-0.2476879954,
-0.0175373573,
-0.021594055,
-0.1326887608,
-0.6107324362,
0.0473336466,
0.2841535807,
0.2572387457,
-0.0001591642,
-0.1271250397,
0.1364337951,
0.3836011589,
-0.0967624336,
0.3567715287,
0.1492610574,
0.036887873,
-0.1886469275,
-0.2704182863,
-0.0527102426,
-0.0406088829,
0.0104832258,
0.1263322383,
-0.0127740772,
0.2184481025,
-0.5251656771,
0.182317555,
0.2948209941,
0.0213304702,
-0.2041355371,
0.1176783964,
0.1039166376,
-0.1102817655,
0.0116426758,
-0.3245984614,
-0.3441009223,
-0.1030216739,
-0.1764565557,
0.209032923,
-0.0325296372,
-0.0658806935,
0.2634501755,
-0.1613494009,
-0.0393637978,
0.4047775567,
-0.4816957116,
-0.1757546514,
0.0467467867,
0.3898822963,
-0.4873673022,
-0.2078987658,
0.7181901336,
0.2839853466,
0.1210317761,
-0.2442062497,
0.0776855573,
-0.2190175653,
0.2853495181,
-0.1448170543,
0.2672016621,
-0.1714928597,
0.1936893612,
0.1794565767,
-0.2558462024,
-0.234559834,
-0.051765535,
-0.0645456761,
0.0557143912,
-0.0814132914,
0.0005702993,
-0.1619116813,
-0.0869019181,
-0.0464038812,
0.2907566428,
0.1483398229,
-0.1066333652,
-0.045483321,
0.199440375,
0.0568395779,
-0.1714216769,
-0.0616391525,
0.2124749571,
-0.1362293661,
-0.1371072382,
0.1286387891,
-0.0290475227,
0.166729793,
0.3370332122,
-0.641823411,
0.2252513468,
0.0984824821,
0.4276833236,
0.2031523436,
0.3347710967,
0.158977136,
0.2815193236,
-0.1367615908,
0.0131743802,
0.2245784402,
0.0837198496,
-0.1395093799,
0.1632851809,
-0.011857694,
0.2165131569,
0.0882183164,
0.1616093367,
-0.0195057709,
0.3819691539,
-0.1094945595,
-0.0760950372,
0.2928076088,
0.1094410345,
-0.1039121225,
0.0777937546,
-0.3227870762,
0.1022258252,
-0.1177079007,
0.1059036851,
0.133849293,
-0.0717743635,
-0.2860381603,
-0.0957184657,
0.2287209183,
0.4395948648,
0.0452026278,
0.2318579257,
-0.2078226656,
0.3169747293,
-0.1538725942,
-0.0106306206,
0.0769629627,
-0.3803690672,
0.3780267835,
-0.1478172839,
0.0793882981,
0.4393112361,
0.4490014911,
-0.1216977313,
0.5643337965,
0.0937559977,
0.1662644744,
0.0777898282,
-0.174787879,
0.0762940571,
0.3394045234,
0.1429420412,
-0.5593454242,
0.0119869048,
0.009836819,
0.0507726222,
-0.0907639414,
0.0733440965,
0.3212529719,
0.0812146887,
-0.1045709178,
-0.0404203944,
-0.003482634,
0.0848081931,
-0.140828222,
-0.0825391337,
0.257242918,
-0.1428915858,
0.2666389644,
-0.2509084642,
0.2829679549,
-0.1334728301,
0.2678283453,
-0.0321185999,
0.3751236796,
0.3795364499,
0.0595999397,
0.2736056447,
-0.1282400638,
-0.0425588265,
0.1863374859,
-0.0076871072,
0.2494966239,
0.3891300261,
0.1700571477,
0.2802571654,
0.0582993999,
-0.0292893779,
-0.1207244322,
-0.0304327253,
-0.0494102389,
-0.067625761,
-0.1022972614,
-0.0696651489,
-0.2004574686,
0.1851495653,
0.1658859551,
-0.3086847067,
0.4326113164,
0.2365029752,
-0.0868011191,
-0.0161571074,
0.1564302295,
0.0106880171,
-0.109747678,
0.0504863113,
0.2927230895,
0.3041375279,
0.1310097426,
-0.5701603293,
-0.1223332211,
0.1272025704,
-0.1752162278,
-0.5535447001,
0.1471072137,
0.0704301521,
0.1053016186,
0.1867406219,
0.1335619241,
0.0324811377,
-0.1700442284,
0.058995191,
-0.0915268213,
-0.1022744253,
0.3059036136,
-0.0794074535,
0.0153195551,
0.1056589782,
0.0515682548,
0.0710837692,
-0.1111140996,
0.0873575956,
-0.2075468749,
0.2392419726,
0.2646926641,
0.249378711,
0.3220413327,
0.6761341095,
-0.1604972035,
0.0959381908,
0.0055759344,
-0.0548029691,
-0.0238488819,
0.1650026739,
-0.0799582824,
-0.3598210216,
-0.301397562,
0.0875920728,
0.3000229299,
0.3268482983,
0.3117436171,
-0.1694518924,
-0.3693900704,
-0.3254944086,
0.0781042576,
-0.2393300086,
0.1011587605,
0.3707355559,
-0.1734389812,
-0.2449707091,
0.0340408944,
-0.1015895903,
0.4346278608,
-0.1382071227,
0.1127894819,
-0.3447737992,
0.1693847775,
0.649116993,
-0.1134444028,
-0.6402677298,
-0.2678225636,
0.348785013,
0.2495370954,
-0.2667504549,
0.1410764009,
0.2826905251,
-0.1879064739,
-0.0119997421,
0.8816472888,
-0.1740210354,
-0.2488285005,
-0.205469504,
-0.0559663139
]
|
https://github.com/huggingface/datasets/issues/1983 | The size of CoNLL-2003 is not consistant with the official release. | We should mention in the Conll2003 dataset card that these lines have been removed indeed.
If some users are interested in using these lines (maybe to recombine documents ?) then we can add a parameter to the conll2003 dataset to include them.
But IMO the default config should stay the current one (without the `-DOCSTART-` stuff), so that you can directly train NER models without additional preprocessing. Let me know what you think | Thanks for the dataset sharing! But when I use conll-2003, I meet some questions.
The statistics of conll-2003 in this repo is :
\#train 14041 \#dev 3250 \#test 3453
While the official statistics is:
\#train 14987 \#dev 3466 \#test 3684
Wish for your reply~ | 73 | The size of CoNLL-2003 is not consistant with the official release.
Thanks for the dataset sharing! But when I use conll-2003, I meet some questions.
The statistics of conll-2003 in this repo is :
\#train 14041 \#dev 3250 \#test 3453
While the official statistics is:
\#train 14987 \#dev 3466 \#test 3684
Wish for your reply~
We should mention in the Conll2003 dataset card that these lines have been removed indeed.
If some users are interested in using these lines (maybe to recombine documents ?) then we can add a parameter to the conll2003 dataset to include them.
But IMO the default config should stay the current one (without the `-DOCSTART-` stuff), so that you can directly train NER models without additional preprocessing. Let me know what you think | [
-0.0790515989,
0.0053056106,
0.0227090288,
0.1767312288,
-0.1750466228,
0.0091939494,
0.19263421,
0.1599833965,
-1.0088421106,
0.0919715613,
0.116121836,
0.0397599377,
-0.0169362314,
0.1187621281,
-0.061569199,
0.3097824752,
0.0787528381,
0.0600118078,
0.147918418,
-0.1191561222,
-0.3082512021,
0.3580761552,
-0.3310670555,
0.0405780524,
-0.5341427326,
0.1651311517,
-0.0984804407,
0.187835902,
-0.4342067838,
-0.4354426265,
0.3668316603,
0.1122713089,
0.4130791128,
0.0164172389,
-0.0001118863,
-0.1192543879,
0.2520218492,
-0.1412217915,
-0.0766935721,
0.169463709,
-0.0502098799,
-0.2738067806,
0.0147661259,
-0.0273160003,
-0.0503354222,
0.2545051277,
-0.109115012,
0.2557536066,
0.0737720951,
0.3681805134,
0.2139770687,
0.0384478793,
0.1702185422,
-0.0514830947,
0.3541508615,
0.2172905952,
-0.0525223278,
0.3811750114,
-0.1951489896,
0.1040109992,
-0.2099632025,
0.2688073516,
0.1873316467,
-0.2268103361,
0.316673398,
0.1540002376,
0.256965369,
-0.2214958519,
-0.2121856064,
0.3500757813,
0.2903464437,
-0.214249149,
-0.1941874474,
-0.2296766192,
0.2725405395,
-0.4574778378,
0.0604044944,
0.0433200225,
-0.0058562751,
0.0137380259,
-0.2138533145,
-0.5553433299,
-0.2926457524,
0.0585917011,
-0.3667202592,
0.3881664276,
-0.1157286763,
-0.1022902429,
0.1817386001,
0.0599914193,
0.6260008812,
0.0103354361,
-0.1692815572,
-0.1431287229,
-0.1197363362,
-0.2859583497,
-0.0559342429,
0.0654562637,
0.2638854086,
-0.0636548549,
-0.1156925932,
-0.4510706365,
0.212199226,
-0.248263225,
-0.0093583865,
0.1131958887,
0.2837645113,
0.2398556322,
0.1120828092,
0.222441256,
0.2796356976,
0.1129841581,
-0.2029008269,
-0.0597664639,
-0.2434740365,
-0.0676077381,
-0.0179156233,
-0.2810792625,
0.1291225255,
0.1988327056,
0.0639457256,
-0.0796380118,
-0.1012123823,
0.0184937716,
0.0820038319,
0.4512065947,
0.0034324941,
-0.1984363943,
-0.0845289007,
-0.3352281749,
-0.1166150421,
-0.2821267247,
-0.082938239,
-0.0459288321,
0.0355485417,
-0.2012433559,
0.1888842285,
-0.1283840686,
0.2089133561,
0.3528968692,
0.1774380207,
-0.1730100513,
0.1446497291,
-0.0073263245,
-0.2975329161,
-0.1950113922,
-0.058565326,
0.1618614197,
-0.2799871862,
0.0485451221,
-0.099347353,
-0.3043543696,
-0.4162813425,
0.1212235466,
-0.1019024923,
0.0118264919,
0.6224349737,
0.166465044,
0.0826452672,
-0.1743581742,
0.0671645254,
-0.4080205262,
-0.3539326191,
-0.1758714616,
0.3193430603,
0.2330729365,
-0.2300451547,
-0.11733412,
-0.0911654457,
-0.150545001,
0.1310711056,
0.0084170485,
-0.1777451783,
0.1105760038,
-0.0720574856,
-0.2215395868,
0.251827389,
-0.3360650837,
-0.3417873383,
0.1112437546,
0.2198237479,
-0.326685667,
-0.0330132544,
-0.0516681299,
0.3488057256,
-0.0335537456,
0.022242859,
0.0991625935,
0.022030931,
-0.2132713199,
-0.3179377317,
-0.2815464735,
-0.3954845369,
-0.1032079384,
0.1473606676,
-0.1974996328,
-0.3529450297,
0.0315566659,
0.3055687845,
0.1924332827,
0.0112374173,
0.3021039367,
0.3747840822,
-0.1904215515,
0.0783938542,
0.2963242233,
-0.6002671719,
0.0333614163,
-0.0880929977,
0.2312198132,
0.7295775414,
-0.0865772292,
-0.048696477,
-0.1075352207,
0.1938240826,
-0.2102125436,
0.1165840924,
0.0602158643,
0.2370425761,
0.0884939134,
-0.1364406794,
0.0785466433,
-0.2363153845,
0.1135210469,
-0.2278852463,
-0.179207772,
-0.0897695944,
-0.0024871181,
0.0092416815,
0.0679096952,
-0.1765298098,
0.2218803912,
0.0440060757,
0.3089011312,
0.3044916987,
-0.3136171103,
0.080999054,
-0.0011138428,
0.007004587,
0.0568198636,
0.0082931034,
-0.2714274228,
-0.3744520247,
0.2555373907,
-0.0846408159,
0.0808003321,
0.4652875066,
-0.0698696226,
0.2097195685,
-0.0009585097,
-0.1454470456,
-0.0171782486,
-0.0766734853,
-0.567127645,
-0.2756134272,
0.3597345948,
-0.0090086926,
0.3806383014,
-0.3556631207,
-0.0670059472,
0.2865485251,
0.0019145668,
-0.410764575,
0.441816628,
-0.3745268583,
-0.08145307,
0.1061327159,
0.4837851822,
0.0947721973,
0.2629714012,
0.2847140431,
0.1773801744,
-0.0606923923,
-0.0977045521,
0.3825394809,
0.0797180012,
0.6006678343,
0.2228207737,
0.015330228,
0.0987113789,
0.087250337,
-0.1637640744,
0.0181886535,
0.1527938992,
-0.2198046297,
-0.2572901845,
0.0601755418,
-0.0677530468,
-0.0747490153,
-0.0177049059,
-0.3243750632,
-0.1396196485,
0.060380023,
-0.2385689914,
0.0713471025,
-0.0294601209,
-0.557931602,
0.3389035165,
-0.2254010141,
0.2155261785,
0.1509422064,
0.0823790953,
-0.5002081394,
0.1482925564,
-0.1069184169,
-0.3531936705,
0.048199676,
-0.4182689786,
0.0668952242,
0.2385120839,
-0.3264963329,
0.3696915209,
0.1347670406,
0.1904295832,
0.2230057865,
-0.3871460855,
-0.1640755385,
0.2841860354,
-0.1811350137,
0.0183177665,
-0.2151916772,
-0.0226862933,
-0.3398991525,
0.2574863136,
-0.1655431241,
-0.4132043123,
-0.0645679012,
-0.1689621508,
-0.0013989165,
0.0684769526,
0.0359239392,
0.0072926064,
-0.0268486496,
-0.0757275745,
-0.400631249,
0.2760812342,
-0.0114549706,
-0.2453077734,
0.2559419572,
0.0025321385,
-0.1818639487,
-0.0200046618,
-0.1705814749,
0.1318050325,
-0.108192876,
-0.6059451699,
0.2363823801,
-0.0703085437,
-0.0106609548,
-0.1274935454,
-0.05979871,
0.3178858459,
0.2325277328,
-0.026087733,
0.0949213356,
0.0383143537,
0.2087804973,
-0.1287232041,
0.4611245692,
-0.0976863652,
0.0773094818,
0.0459612906,
0.3247405887,
0.2846694291,
-0.2928981185,
-0.1263490021,
0.0874622315,
0.3358828425,
0.0002201374,
0.2209351361,
0.0259131845,
0.0353936553,
0.0152605986,
0.3652921021,
0.2062508613,
-0.115199931,
0.017417632,
0.2381313592,
0.1925429106,
-0.1675613374,
0.032713566,
0.2342021912,
0.6284462214,
-0.1660372466,
0.0329458117,
0.0276788175,
-0.3331249952,
-0.1734183431,
-0.1224723309,
0.1904444098,
-0.1301647872,
0.1776046306,
0.5518294573,
-0.5440957546,
0.2639869452,
-0.0514189899,
0.0805386603,
0.1151378676,
-0.2817249,
-0.1604717225,
-0.0060485974,
0.4679948688,
-0.0481593348,
-0.412579298,
0.3504716754,
0.1653052568,
-0.4734294415,
-0.1215543151,
-0.2587653995,
-0.5339021087,
1.0868718624,
0.5440802574,
-0.0359398648,
-0.2314253449,
0.3389776349,
-0.0286156572,
-0.222127229,
-0.1624897271,
-0.2948036492,
0.0752848908,
-0.0209757704,
-0.055056747,
-0.2603351176,
-0.1130759344,
-0.2074434608,
-0.0102988863,
0.2051172256,
-0.1974100918,
0.0964839086,
0.219110176,
0.3813681602,
0.1402321905,
0.2431928217,
0.0593852401,
0.4666585922,
0.4851014018,
0.1532858014,
-0.0573613271,
-0.2719488442,
0.2822734118,
-0.1091230363,
0.5299078822,
0.3107829392,
-0.0572633632,
-0.021301765,
-0.2038783431,
0.0771392435,
0.0760961622,
0.2159231454,
0.3242282271,
-0.2165441662,
-0.3204388618,
-0.1204322129,
0.0201633833,
0.2061260045,
-0.202785179,
-0.4543351233,
-0.1485805362,
-0.0730137303,
-0.1013199463,
0.4920628965,
0.8532764912,
0.0660226271,
0.2991862297,
-0.0171368513,
-0.2874809802,
0.4888044894,
-0.0867124349,
0.1391885728,
-0.3684086204,
-0.0079656066,
0.0710504949,
-0.1088033766,
-0.1466743052,
0.0864087939,
-0.1399548948,
0.0994460583,
-0.1318168342,
-0.2483180016,
-0.0121793607,
0.3627191782,
0.0624586903,
0.0470756255,
0.6486375332,
0.0402391367,
-0.0018704593,
-0.1850621998,
-0.0330131911,
-0.0668509081,
-0.1162804738,
0.0411882587,
-0.1368859559,
-0.1115400568,
0.0805256665,
-0.0213482175,
0.1995276958,
-0.4030070603,
0.0898967162,
0.5373718143,
0.1734156311,
0.2524739206,
-0.2667485178,
-0.0780734867,
0.2593310773,
-0.1200408116,
0.398304075,
0.13484855,
0.0571174547,
-0.2200692892,
-0.2907458246,
-0.0389301553,
0.1188781187,
0.1148884594,
0.0022324594,
-0.3247586191,
0.1397278011,
-0.5065875053,
0.141708374,
0.070765622,
0.0411732085,
-0.1231541559,
0.2083689868,
-0.1243160889,
-0.0224425849,
0.0308007766,
-0.1415689886,
-0.3870671391,
-0.0869080275,
-0.1063448936,
0.2709775269,
0.2768913209,
0.127536118,
0.149686113,
-0.0527112335,
-0.0856911317,
0.2901490331,
-0.1613325626,
-0.1506537199,
0.1519983113,
0.4072240591,
-0.0559114479,
-0.0959187895,
0.2289099544,
0.2441459149,
0.0138012581,
-0.0843922123,
0.2946996987,
-0.4281078875,
0.1821243763,
-0.2010682523,
0.4821614027,
-0.1125019714,
-0.0547958426,
-0.0732146278,
-0.2601737976,
-0.3238525987,
-0.0153864138,
-0.197471559,
-0.0878955275,
-0.2802775204,
0.0168674048,
-0.2452735603,
-0.0561282411,
0.0320811346,
-0.0878541097,
-0.0835088119,
-0.1851974428,
-0.0691231191,
0.1099172384,
-0.0350423604,
-0.1135482714,
0.1861982793,
0.098731555,
-0.3318323791,
-0.0568742827,
-0.0724850073,
-0.2056240141,
0.2165593505,
0.6052778959,
-0.4788678885,
0.0174341816,
0.2167271674,
0.3572168648,
0.0631685853,
0.2910677493,
0.1918245554,
0.5138147473,
-0.0972713456,
0.0167564396,
-0.0803731903,
0.139407292,
-0.3710765541,
-0.037503574,
-0.0178774167,
0.0828926489,
-0.0480665714,
-0.0041853758,
0.1387232095,
0.1144267842,
-0.0810979232,
-0.1132285073,
0.2425559312,
0.2390531003,
-0.2424699068,
0.0384461358,
-0.1725451499,
0.0587646998,
-0.0347019546,
0.1650695652,
0.1652334034,
-0.0666310862,
-0.1837199926,
0.150230512,
-0.0941495448,
0.4256551564,
-0.0145785026,
0.243922025,
0.0069968873,
0.3048892617,
0.3469515145,
-0.129991591,
0.15805538,
-0.0452575088,
0.5192873478,
-0.0432185307,
-0.1320543587,
0.3089759946,
0.3415549994,
-0.4437061548,
0.4573958516,
0.1989683211,
0.1121174246,
0.0746016204,
-0.0678191707,
0.0970050842,
0.4011939764,
-0.1341478974,
-0.5298086405,
0.027815897,
-0.0927998275,
-0.176802218,
-0.1649042219,
-0.0588299632,
0.4024438858,
0.3872848153,
-0.060808219,
-0.1517053097,
-0.0667163432,
0.092359215,
-0.2444108874,
0.0574365892,
0.5005825758,
-0.0221062656,
0.0581258871,
-0.2497322559,
0.0316068083,
-0.112710461,
-0.0047118841,
-0.1360365897,
0.4472353458,
0.2742930353,
0.0504243076,
0.1626671851,
-0.1984321326,
0.2732846141,
0.0497829616,
-0.1446202844,
0.1420492977,
0.5019463897,
0.2180439383,
0.1841220409,
0.1261109263,
-0.0163301174,
-0.2222192734,
-0.1227296963,
0.0380655527,
-0.0254142061,
0.1366608739,
0.24366045,
-0.1785572469,
0.1551505625,
0.2969260812,
-0.4654336274,
0.3182476461,
0.5749579072,
0.1311012059,
0.0354702249,
0.0578069836,
0.0711148754,
-0.1768958569,
0.0446702056,
0.2089404166,
0.1623998731,
-0.1082177162,
-0.5213891864,
-0.0811595023,
0.2729400694,
-0.1001614332,
-0.1645810604,
0.131347537,
0.1835099459,
0.0777962208,
0.0132115744,
-0.1120466292,
-0.0527080894,
-0.438475728,
0.1809065193,
-0.1560252607,
0.0202434883,
-0.1199272275,
-0.0052764267,
0.0289471,
-0.1149221659,
-0.0118541978,
-0.1289932132,
-0.0810680538,
-0.0968910158,
0.1312232912,
0.3844471276,
0.4371201992,
0.2869356871,
0.2150787711,
0.3534070253,
-0.0599928312,
-0.2232438177,
-0.0786948502,
0.2101554573,
-0.2013533115,
-0.0585583337,
-0.2093857229,
-0.2135192305,
-0.3199555278,
-0.1325806677,
0.1056051031,
0.2887851,
0.2079715133,
-0.0856633708,
-0.3484726846,
-0.145023033,
0.1012751833,
-0.261371702,
0.2602858543,
0.2764099538,
-0.1039938033,
-0.2661340535,
-0.0656883046,
-0.0074326741,
0.3319245577,
0.0968860462,
0.2977833152,
-0.4783822894,
-0.0235061385,
0.2744100392,
0.0364439711,
-0.4960816503,
-0.07097096,
0.4799347222,
0.134875536,
-0.2606061697,
0.3722866476,
0.1307561547,
-0.0844979584,
-0.1351377517,
0.5800475478,
-0.3409999609,
-0.349898845,
-0.5202121139,
-0.1578115374
]
|
https://github.com/huggingface/datasets/issues/1983 | The size of CoNLL-2003 is not consistant with the official release. | @lhoestq Yes, I agree adding a small note should be sufficient.
Currently, NLTK's `ConllCorpusReader` ignores the `-DOCSTART-` lines so I think it's ok if we do the same. If there is an interest in the future to use these lines, then we can include them. | Thanks for the dataset sharing! But when I use conll-2003, I meet some questions.
The statistics of conll-2003 in this repo is :
\#train 14041 \#dev 3250 \#test 3453
While the official statistics is:
\#train 14987 \#dev 3466 \#test 3684
Wish for your reply~ | 45 | The size of CoNLL-2003 is not consistant with the official release.
Thanks for the dataset sharing! But when I use conll-2003, I meet some questions.
The statistics of conll-2003 in this repo is :
\#train 14041 \#dev 3250 \#test 3453
While the official statistics is:
\#train 14987 \#dev 3466 \#test 3684
Wish for your reply~
@lhoestq Yes, I agree adding a small note should be sufficient.
Currently, NLTK's `ConllCorpusReader` ignores the `-DOCSTART-` lines so I think it's ok if we do the same. If there is an interest in the future to use these lines, then we can include them. | [
0.1301076263,
0.0962899998,
0.0394510366,
0.0533670262,
-0.2169310898,
0.0075206906,
0.1542738676,
-0.0001620095,
-0.9731167555,
0.0672689676,
0.20561257,
0.0701418817,
-0.0513290241,
-0.0842716619,
-0.0803269222,
0.3419830203,
-0.0675748587,
0.3278605044,
0.2285469472,
-0.1227637231,
-0.366845876,
0.2775171697,
-0.2749780118,
-0.0082391109,
-0.393465817,
0.0806121603,
-0.1419338435,
0.2710109949,
-0.2415266484,
-0.5320182443,
0.3974418938,
0.2227788419,
0.0772374868,
0.0288813449,
-0.000115512,
-0.2126497626,
0.3872513175,
-0.2159655243,
-0.2099746764,
0.4730772078,
-0.1129044369,
-0.7660292983,
-0.0443733409,
-0.4447827935,
0.0319345817,
0.5121639371,
-0.081752196,
0.1743455082,
0.2542612851,
0.1613585651,
0.1676935554,
0.239318192,
0.2375085205,
0.1333179325,
0.3475007415,
-0.0249684937,
-0.1696095914,
0.550845623,
-0.2081133276,
0.1203337759,
-0.2780005038,
0.3548775315,
0.1467531919,
-0.2336142659,
0.1369725317,
-0.0380337052,
0.2645011544,
-0.0674745813,
-0.1419987977,
0.290950954,
0.2943903804,
-0.2650745213,
-0.1194989383,
-0.5421313047,
0.2677497566,
-0.4985672235,
0.099537909,
-0.0365588404,
-0.0151118059,
0.0170590542,
-0.2545986176,
-0.419888109,
-0.3282956183,
0.1200166717,
-0.1598797441,
0.418084085,
-0.0272402354,
0.0301429275,
0.1538592428,
0.1586637795,
0.5796191096,
0.0767197236,
-0.244494468,
0.0215664543,
-0.0377821922,
-0.4418599308,
0.1902303398,
-0.1309908628,
0.1642339975,
-0.2104474306,
-0.1204380244,
-0.4199703634,
-0.0901066959,
-0.0593100041,
0.0193503667,
0.0543407761,
0.4128797948,
0.3364708722,
0.2408224344,
-0.1000609696,
0.4114395678,
0.143622309,
-0.2529670298,
-0.1811208725,
-0.1239448264,
0.1675827652,
-0.2642976642,
-0.2406896055,
0.162481457,
0.1565831453,
-0.034936402,
-0.0735282451,
-0.0618178174,
-0.0178563669,
0.2747398317,
0.3580441773,
0.0686172172,
-0.0945586339,
0.0771590322,
-0.2723612189,
-0.0594633371,
-0.3487529755,
0.005908126,
-0.0171535648,
-0.1843848228,
-0.0562592372,
0.3026265204,
-0.3127998114,
0.2598235309,
0.3169445693,
0.1503163278,
0.0124025596,
0.0943747833,
0.0488492437,
-0.0882599577,
-0.1484765559,
0.0212387629,
0.1710013449,
-0.3730175197,
0.2206998467,
0.1078786775,
-0.3871751428,
-0.4981612265,
0.0574467555,
-0.3294163644,
-0.0661146119,
0.5692336559,
0.0615519285,
0.1533861011,
-0.2984738052,
0.2479479462,
-0.1679145098,
-0.1764442325,
-0.1377007514,
0.2795293331,
0.0724272355,
0.077220045,
-0.2327022254,
-0.2192711681,
-0.1420801431,
0.2104844302,
0.0464700311,
-0.1911342889,
0.1837271452,
-0.0846437961,
-0.0169069432,
0.4908644557,
-0.3189496398,
-0.2493044138,
0.4301477373,
-0.0368910879,
-0.2194399834,
-0.0988786817,
0.1389303505,
0.1706577241,
-0.1958432645,
-0.0332032368,
0.1517600715,
0.0310925171,
0.1289449334,
-0.3339414597,
-0.2219436616,
-0.2317957729,
-0.2167308033,
0.1633665413,
-0.2227857709,
-0.2178170532,
0.2956934273,
0.4338990152,
0.0595171526,
-0.0693796724,
0.2499161065,
0.3685536087,
-0.2128673792,
-0.1087666973,
0.4909599721,
-0.3863347173,
0.0884430483,
0.0807879418,
0.2146431953,
0.7478346825,
-0.2653278112,
-0.230581522,
-0.1599821299,
0.2598364353,
-0.003771964,
0.110420242,
0.1521412581,
0.1595449895,
0.231408596,
0.1487795711,
0.0730552375,
-0.1615211219,
0.037176121,
0.0772234797,
-0.185334444,
-0.0302613731,
-0.1165649891,
0.1630455405,
0.3133792877,
0.0409842879,
0.2251079232,
-0.1252538711,
0.3692484498,
0.0459582619,
-0.0481309034,
0.0709814057,
-0.1661588401,
0.0461776219,
0.2672272921,
-0.0127219111,
-0.3932290077,
-0.4152543545,
0.0613913126,
0.0116803916,
0.0987936929,
0.5511186719,
-0.2438949049,
0.0746926516,
-0.084113054,
-0.1902332902,
-0.1499232948,
-0.2079463452,
-0.2950228453,
-0.2136442065,
0.3231473267,
-0.2128849477,
0.1534338593,
-0.31016922,
-0.0633997172,
0.4416496754,
-0.1853234619,
-0.1847078204,
0.3180697858,
-0.2418947369,
-0.0890743509,
0.248134762,
0.2256138623,
0.0159508027,
0.2709317803,
0.2830391228,
0.1357546747,
-0.2408513874,
-0.1283156723,
0.1752320379,
0.2388230562,
0.439444989,
0.2391956598,
0.0922867581,
0.1148137674,
0.0340717435,
-0.0045290468,
-0.0054155742,
-0.0277315211,
-0.2279876024,
-0.3621946871,
0.1602540761,
-0.1504976749,
-0.065082103,
-0.3093796372,
-0.2254503965,
-0.3511162996,
0.1018533036,
-0.138807416,
-0.1369156539,
0.1145196855,
-0.5290245414,
0.4189196527,
-0.0392220616,
0.2031293809,
0.2047450244,
-0.2497672439,
-0.5104730725,
0.085344933,
-0.0410053954,
-0.3061436713,
0.3523703218,
-0.4794407785,
0.0280888714,
0.2486196905,
-0.4806946516,
0.3634322882,
-0.0830030739,
-0.1581347734,
0.2018321604,
-0.2051296532,
-0.3833320439,
0.1208055764,
-0.2449854165,
0.2246064842,
-0.1539994031,
0.0005650504,
-0.072046265,
0.212302655,
-0.4482310712,
-0.1638088375,
0.1669666767,
-0.3378915787,
0.1977344155,
-0.0912566856,
0.0160745475,
0.0817924514,
-0.1622833461,
0.201184541,
-0.1818698198,
0.1154795289,
-0.1136729121,
0.0167074595,
0.2259346396,
-0.0811314657,
-0.1662235856,
0.0699584186,
-0.2387209237,
0.0106034651,
-0.307862401,
-0.7261505127,
0.0743751898,
0.0595257543,
-0.0601581335,
-0.1150821671,
0.037605118,
0.2131721973,
0.1127523929,
0.0523195565,
-0.0855743438,
-0.122623533,
0.1621291488,
0.0945108607,
0.5319266915,
-0.2228879184,
0.3096297979,
-0.0022928854,
0.1590560228,
0.2853764296,
-0.0007306093,
-0.2252565622,
-0.0015947262,
0.2930029631,
0.2588473856,
0.3286584318,
0.3251233697,
0.1030991673,
-0.137955606,
0.4538410902,
0.0774001852,
-0.1460178643,
0.0553969108,
0.1441565603,
0.0169384368,
-0.4341698289,
0.0573548824,
0.1168069467,
0.5626437664,
-0.2184776217,
-0.0084213475,
-0.1097343713,
-0.1650134325,
-0.2341182232,
-0.0293199979,
0.3005229831,
-0.0291776247,
0.2054884881,
0.2458188534,
-0.5631028414,
0.2150886059,
-0.0362780653,
0.0829903036,
0.1746260971,
-0.2535677254,
-0.2356996834,
0.1044341177,
0.0360957421,
-0.0938178152,
-0.206331104,
0.3459341824,
0.3252568841,
-0.2910983562,
-0.2175761461,
-0.2208031565,
-0.2699078619,
1.2819191217,
0.4255767763,
-0.3862963021,
0.0430356413,
0.3762326539,
-0.1483178139,
-0.1491272748,
-0.2190727592,
-0.3982901871,
-0.1763947308,
-0.1942517459,
0.1178859696,
-0.0154518634,
-0.0890325978,
-0.1234605685,
0.0912795588,
0.3205406964,
-0.1780039668,
0.096841678,
0.3929636776,
0.2283859998,
0.5601587892,
0.1672638208,
0.0511209667,
0.318878144,
-0.0553445332,
0.1946553886,
0.1176549643,
-0.0828343779,
0.2511395812,
-0.3525597155,
0.4620983601,
0.3488355875,
0.1313163936,
-0.1007163152,
-0.1621300429,
0.0177412368,
0.1748204231,
0.3541528881,
0.1557819098,
-0.3248018324,
-0.3251656592,
-0.1433885545,
0.2663402557,
0.0685090721,
-0.1487400383,
-0.4119541943,
-0.1902712733,
-0.2139977962,
-0.1538047791,
0.2392158508,
0.8080552816,
0.1239834055,
0.1704270691,
0.3559843898,
-0.2942590415,
0.4923354685,
0.0814509466,
0.0388295762,
-0.3318096399,
0.2694885731,
0.1430428624,
-0.0412596017,
-0.246694684,
-0.0483896323,
-0.4165377021,
-0.0031494675,
0.0022389542,
-0.2413030416,
-0.0930721462,
0.3237604797,
0.05278413,
0.0639534071,
0.2752558291,
0.038937442,
0.1326100826,
-0.0510024801,
0.0120957643,
-0.2595163286,
-0.1758070737,
-0.1770465672,
-0.353235513,
-0.3114776909,
0.1412599087,
0.0185090136,
-0.0236559212,
-0.4095527232,
0.1921206564,
0.277020365,
0.3589101732,
0.2970503569,
-0.2169284075,
-0.1093113571,
0.2528964877,
-0.2034263611,
0.3574486971,
0.2469754368,
0.0348186344,
-0.2830647528,
-0.1956482232,
0.1517183185,
-0.1001771241,
-0.0376819558,
0.1460777223,
-0.1781590581,
0.0142082255,
-0.3417794406,
-0.0354530364,
0.3411012888,
-0.0877544731,
-0.0100297946,
0.1375861019,
0.0932519957,
-0.0353013761,
-0.1563231498,
-0.2820818424,
-0.2060043514,
-0.0444565564,
-0.3939486742,
0.137253955,
0.1824113578,
-0.0218910109,
0.0423905477,
0.0228500385,
0.0014151909,
0.1289570928,
-0.4131377041,
-0.0770954192,
-0.066857338,
0.5177925229,
-0.2952236235,
-0.2913728952,
0.248176083,
0.1947961897,
0.2999886274,
-0.1022826657,
0.2632978857,
-0.4071255028,
0.2513201535,
-0.2720457613,
0.445905596,
0.0000088875,
0.4026787579,
-0.0745482892,
-0.1872903109,
-0.2772980034,
-0.2720987201,
-0.2297483981,
0.0107980696,
-0.187949568,
0.0458861738,
-0.145642966,
-0.1231526062,
-0.0274069328,
0.1666629016,
-0.0020090402,
-0.1085649058,
0.0261016302,
0.1678994596,
0.0458098203,
-0.036275398,
0.2290016413,
0.0017383397,
-0.1395779997,
-0.020596249,
0.039450109,
-0.3833729029,
0.1314447075,
0.6604418755,
-0.5886904001,
0.4171709716,
0.2511738539,
0.2564397454,
-0.0192746203,
0.3532113135,
-0.1148338914,
0.542820096,
-0.0046753995,
0.1038531512,
0.2478197515,
0.065843232,
-0.3922306895,
0.1441471577,
-0.0034273786,
0.1552132517,
-0.0760343149,
0.0041326,
-0.0537704602,
0.0722349435,
-0.2965032756,
-0.199016273,
0.2163129002,
0.1753439903,
-0.2990160882,
0.1887125671,
-0.1672665924,
-0.034873683,
0.1587670445,
0.1492213756,
0.0316301771,
-0.1084924713,
-0.1870096773,
0.2079919875,
-0.1151562184,
0.389423877,
-0.0186804943,
0.2622986436,
-0.0982709751,
0.2752375305,
0.0979675725,
-0.132003054,
0.2643316388,
-0.4030515552,
0.5268943906,
-0.1661984324,
-0.1249704063,
0.2696354091,
0.184337765,
-0.2103803754,
0.3639317155,
0.3102309704,
0.0992765427,
0.2528161108,
0.0409559011,
0.1101133898,
0.1543637365,
0.1772451997,
-0.6114675999,
0.1304256618,
-0.0217847638,
0.0371778607,
0.0025649997,
0.2486211509,
0.197456643,
0.1135745198,
-0.0705643892,
-0.0309345406,
-0.3614553213,
0.0356949158,
-0.0779793561,
0.2171178013,
0.335872978,
0.1498037726,
0.0945752263,
-0.3691617846,
0.0371610746,
-0.1639919579,
-0.0264077932,
-0.1165777668,
0.4230067432,
0.3049896657,
0.0142474519,
0.1797240674,
-0.0623153038,
0.1979971826,
0.1574594527,
-0.1397769153,
0.0717099011,
0.3436504006,
0.0809429586,
0.2746039331,
0.1012331918,
-0.0663010404,
0.0407200828,
-0.1917509139,
-0.1818829924,
0.0257629715,
0.1480091959,
0.1553257704,
-0.2714480758,
0.1351746619,
0.1511494368,
-0.274874568,
0.3268389106,
0.3695693612,
0.3335462809,
0.0922206491,
-0.1199247316,
0.0460597649,
-0.0518143028,
0.1357114464,
0.3351660967,
0.1156701222,
0.0591662787,
-0.647233963,
-0.2615150511,
0.2753871083,
-0.1858986616,
-0.6451839209,
0.3044980764,
0.0233300179,
0.1042954326,
0.0785891861,
-0.1120034233,
-0.1032510251,
-0.4663709998,
-0.1224791929,
-0.1709789336,
-0.1859330982,
0.0332652628,
-0.0808857903,
0.0616830662,
-0.0555323623,
0.089419283,
0.0845134854,
-0.0057156915,
-0.0798986554,
0.1564452052,
0.4028078616,
0.508806169,
0.2772643268,
0.193552807,
0.5034734607,
-0.1609891504,
-0.2187091261,
0.0961424261,
0.0153531069,
-0.0406199023,
-0.1719724983,
-0.2326990068,
-0.0901061743,
-0.2025837451,
0.1903230995,
-0.0134503692,
0.2978093326,
0.2321576625,
-0.0934982449,
-0.1128151417,
-0.1320216209,
-0.0002958692,
-0.1756173223,
0.0633368716,
0.2933473587,
-0.0990401208,
-0.0328287371,
-0.0693779662,
-0.2204185873,
0.2743247151,
-0.095502384,
0.1714578569,
-0.2696418166,
0.1478173733,
0.0846033245,
0.1020461246,
-0.5734165311,
-0.0021996733,
0.4456372261,
0.3907031119,
-0.3774710298,
0.2812389135,
0.1921704412,
-0.1185682565,
0.031698022,
0.6229762435,
-0.2807606161,
-0.5063766837,
-0.4081285894,
-0.2519993484
]
|
https://github.com/huggingface/datasets/issues/1983 | The size of CoNLL-2003 is not consistant with the official release. | I added a mention of this in conll2003's dataset card:
https://github.com/huggingface/datasets/blob/fc9796920da88486c3b97690969aabf03d6b4088/datasets/conll2003/README.md#conll2003
Edit: just saw your PR @mariosasko (noticed it too late ^^)
Let me take a look at it :) | Thanks for the dataset sharing! But when I use conll-2003, I meet some questions.
The statistics of conll-2003 in this repo is :
\#train 14041 \#dev 3250 \#test 3453
While the official statistics is:
\#train 14987 \#dev 3466 \#test 3684
Wish for your reply~ | 30 | The size of CoNLL-2003 is not consistant with the official release.
Thanks for the dataset sharing! But when I use conll-2003, I meet some questions.
The statistics of conll-2003 in this repo is :
\#train 14041 \#dev 3250 \#test 3453
While the official statistics is:
\#train 14987 \#dev 3466 \#test 3684
Wish for your reply~
I added a mention of this in conll2003's dataset card:
https://github.com/huggingface/datasets/blob/fc9796920da88486c3b97690969aabf03d6b4088/datasets/conll2003/README.md#conll2003
Edit: just saw your PR @mariosasko (noticed it too late ^^)
Let me take a look at it :) | [
-0.1177798361,
-0.1490900367,
-0.1239805967,
0.4063590765,
-0.1421679258,
-0.1860253215,
0.2695666254,
0.010811206,
-0.9765857458,
0.1297612935,
0.0317088179,
-0.0465300158,
0.0295427423,
0.1740382761,
-0.07360439,
0.1277455688,
0.0628561154,
-0.1194889843,
-0.0711035579,
-0.1619691849,
-0.1469118744,
0.4227977693,
-0.3452403247,
-0.0569807179,
-0.4710492492,
0.2424958646,
-0.1226275861,
0.070117645,
-0.5567561984,
-0.3233382702,
0.4087006748,
-0.0866103545,
0.1769327223,
0.3554419577,
-0.0001040467,
-0.1748576462,
0.2246980667,
-0.1157424375,
-0.0655558929,
0.2404019088,
-0.2004333138,
-0.1920389831,
-0.1547531039,
-0.0302859582,
0.0281113628,
0.2732363343,
-0.3089978993,
0.299392283,
0.18221654,
0.2430880219,
0.2945326865,
0.0809209943,
0.2179149985,
-0.1760446429,
0.2989800572,
0.1917586178,
-0.1165723056,
0.2260448337,
-0.1307203025,
0.2275835723,
-0.0872835442,
0.286042273,
0.334974587,
-0.1724303663,
0.0849061832,
-0.0261915736,
-0.0870816037,
-0.0864437819,
0.0954534784,
0.3211068213,
0.3949130774,
-0.312178582,
-0.4026528895,
-0.191369012,
0.031051822,
-0.3462637365,
0.0563205816,
0.009638614,
0.046132043,
-0.0584370159,
-0.4982581735,
-0.1443537325,
-0.2075567096,
-0.0425584279,
-0.3624975681,
0.4512240887,
-0.2420071065,
0.0177785717,
0.1008714214,
-0.0527408756,
0.4142460525,
0.0274848808,
-0.092244558,
-0.0860949531,
-0.2407748103,
-0.3211271465,
0.1106195748,
-0.1267757565,
0.413469553,
-0.1761906147,
-0.3213568032,
-0.4195230305,
0.0159682333,
-0.3341850638,
0.1879398227,
0.2340656221,
0.0104158204,
0.1668917835,
0.1190469936,
0.0761190206,
0.2723382711,
0.0930756927,
-0.2449100614,
-0.1001407877,
-0.2591288388,
-0.0199950207,
-0.1423549205,
-0.2868363261,
-0.1039167941,
0.0991117954,
-0.0918711126,
-0.1583968699,
-0.046987541,
0.1526240557,
-0.007952447,
0.4125003517,
-0.1267407238,
-0.0555191487,
-0.0259354077,
-0.2938324809,
-0.2362735718,
-0.3069846928,
-0.1196085662,
-0.2069771737,
-0.1334819198,
-0.1495283395,
0.2941319048,
-0.1133823395,
0.2720678747,
0.2048838437,
0.0209800079,
-0.1310986429,
0.0350975394,
-0.0036285615,
-0.2124439776,
-0.2451636195,
-0.0987365916,
0.1680229753,
-0.2560645938,
-0.1284076571,
0.0571402423,
-0.324976325,
-0.3823206723,
0.1705316156,
-0.1979278326,
-0.0290266611,
0.5316365957,
0.0959366858,
0.0505766794,
0.1557333469,
0.1403922737,
-0.3408175409,
-0.069907628,
-0.2292313725,
0.3176359534,
0.0836291611,
-0.1907850355,
-0.07872013,
-0.0876960233,
-0.2094605118,
0.1775776148,
0.2158051282,
-0.0621551722,
-0.0934579894,
-0.108381018,
-0.2343146801,
0.1496349573,
-0.3616793752,
-0.4399794042,
0.2209205925,
0.1757687777,
-0.2946115136,
0.0770808831,
-0.0218826123,
0.3208504021,
0.0463332832,
0.0911386311,
0.0918139443,
0.0417531431,
-0.0348821655,
-0.1790224314,
-0.3428453207,
-0.3542280495,
-0.1385771781,
0.2041585892,
-0.3445739448,
-0.1481055021,
0.0730544627,
0.3761892617,
0.2262467295,
-0.0707724392,
0.2620156705,
0.496468991,
-0.1420651972,
0.0202059317,
0.2618137896,
-0.3479644358,
0.0389451683,
0.1203961745,
0.1105895042,
0.7259975076,
-0.1705369204,
-0.2706594169,
0.0465807579,
0.249364838,
-0.0999447331,
0.2031152546,
0.0404589176,
0.3650391698,
-0.0040920083,
-0.1104713753,
0.1139744148,
-0.0178986266,
-0.0292758327,
0.1010705754,
-0.137058109,
-0.0016142796,
-0.0653422326,
0.1549782753,
0.2403029799,
-0.0411919616,
0.1002098918,
-0.0479529649,
0.2280958593,
0.301214993,
-0.2031024396,
0.2046183646,
0.0422944948,
0.0624804795,
0.2254554331,
0.0878700688,
-0.4247790277,
-0.2324255407,
0.2775833309,
-0.1241129413,
0.2256474346,
0.4845543802,
-0.2323308587,
0.1195513457,
-0.0293783918,
0.0251129027,
-0.0848060101,
0.0052103722,
-0.3428602517,
-0.220459342,
0.1815627366,
0.0575423874,
0.2655124664,
-0.3689698279,
0.0382845104,
0.3136284053,
-0.0431070514,
-0.3063028455,
0.2688685954,
-0.4913648069,
-0.0822649524,
0.2280890644,
0.4648599327,
0.1076149344,
0.2788804471,
0.4252998829,
0.0682945997,
0.051812984,
-0.0445775874,
0.1996300966,
0.0479051881,
0.4186435938,
0.143641144,
0.0831094012,
0.0965784565,
-0.0389879979,
-0.0646343082,
-0.0050438303,
0.0042767059,
-0.0824386403,
-0.242688477,
-0.0907875001,
-0.0100193545,
-0.0121672545,
-0.217655912,
-0.2514375448,
-0.1626990736,
0.1214176044,
-0.3009019196,
0.051075723,
-0.0633104369,
-0.3588638604,
0.1681368351,
0.0136897182,
0.313544035,
0.2385149747,
0.1388935298,
-0.4799479544,
0.2259169817,
-0.1357045174,
-0.3781637847,
0.2379620373,
-0.416647166,
0.0695395991,
0.0819516107,
-0.2997786105,
0.3862541914,
-0.1278281957,
0.0064580324,
0.1638872921,
-0.3423286378,
-0.2572751641,
0.3563470244,
-0.0730230063,
-0.1017332077,
-0.2386433035,
0.0057297028,
-0.3039767146,
0.0857307166,
-0.2400318384,
-0.2603769302,
-0.0834303796,
-0.0932430327,
0.1943805963,
0.0187603142,
-0.0278613344,
0.0240292232,
0.1147528291,
0.157236591,
-0.2134674937,
0.1495198756,
-0.2803866565,
-0.3806750476,
0.1139083132,
-0.0351744704,
-0.2225521207,
0.0481996946,
0.0305068307,
0.0931164473,
-0.1576382369,
-0.6883490682,
-0.088828437,
-0.1576086432,
0.1290766299,
-0.1252104491,
0.155696705,
0.2230455875,
0.0539969653,
-0.1211584136,
-0.1104232296,
-0.1563751996,
0.041127108,
0.0945948437,
0.6660491824,
-0.1945739686,
0.2059248835,
0.243672803,
0.4627051353,
0.4733082354,
-0.2580981255,
-0.0867399797,
-0.0067995335,
0.2599476278,
0.012500219,
0.3047553897,
0.3487645686,
-0.0693453923,
-0.0428561568,
0.3592761755,
0.2311931401,
-0.0112244245,
0.1118726283,
0.2965574265,
0.1082372367,
-0.0736091882,
-0.0059639979,
0.4028125703,
0.4447232783,
-0.1062419042,
0.1473759115,
-0.1348290443,
-0.1046176776,
-0.1210753322,
-0.2605170906,
0.0995493606,
-0.1071475819,
0.2515937686,
0.3448479474,
-0.6651978493,
0.3250332475,
0.0519772694,
0.0264490563,
0.0646593347,
-0.1042146608,
-0.1022303104,
0.084546797,
0.40170753,
0.0158035457,
-0.1736896932,
0.2601075172,
-0.0285712555,
-0.3924729526,
-0.0987122655,
-0.4133392572,
-0.418615967,
1.0134066343,
0.4779087901,
-0.1989346743,
-0.0723821148,
0.2843050957,
0.1422546655,
-0.0698137879,
-0.1559869647,
-0.3303982913,
-0.1588705033,
0.0416566804,
0.0585857332,
-0.0234087743,
0.0060524065,
-0.1393435448,
-0.1141657531,
0.2211966217,
-0.2400443852,
0.0303375274,
0.2552056909,
0.4171556532,
0.2711315751,
0.1991782188,
-0.0221704599,
0.359005183,
0.2364827543,
0.387263149,
0.0796740279,
-0.2558591068,
0.3316527903,
-0.0051724389,
0.4434926212,
0.3573232293,
-0.0277479794,
-0.180855751,
-0.2613324821,
0.1665478796,
0.0823950619,
0.2123360038,
0.3883031905,
-0.0115758926,
-0.2462442368,
-0.1123891026,
0.1828770339,
0.1039712504,
-0.0659532472,
-0.2461930066,
-0.0813807249,
0.0203254409,
-0.0806614235,
0.2885064185,
0.8127253056,
-0.0819906145,
0.0596106052,
0.0411754586,
-0.3376260698,
0.2398926467,
-0.0695429817,
0.1148762032,
-0.3390149176,
-0.0835398063,
0.1362097263,
-0.1137317494,
-0.2418110073,
-0.0780687854,
-0.1553236842,
0.0390688181,
-0.1424755901,
-0.0921166167,
-0.0923302621,
0.2410523593,
-0.0133184334,
0.1570476294,
0.4033717215,
0.2571754456,
0.2467218637,
0.0312090218,
-0.0076870983,
-0.0942279547,
-0.2511578798,
-0.0310156085,
-0.450699985,
-0.1496547312,
-0.0415389799,
0.0437066369,
0.1463959217,
-0.4477828145,
-0.0523126423,
0.4075406492,
0.1918008327,
0.1762990355,
-0.1778765321,
0.1143678874,
0.1589151323,
0.1622077227,
0.4319051504,
0.2148407996,
-0.1464107782,
-0.1885229647,
-0.2969200015,
0.1371773183,
0.0668580011,
0.0508466065,
0.062093284,
-0.1832194179,
0.0084642479,
-0.4938531518,
0.3007801771,
0.0256840773,
0.0652870685,
-0.0152885178,
0.2463905066,
0.0747113153,
-0.0519327819,
0.0346722715,
-0.0704205111,
-0.4362964332,
-0.1606467068,
-0.1092336327,
0.1591437161,
0.3684763014,
-0.0709741116,
0.1098432839,
0.0327449664,
-0.0309940297,
0.2632470131,
-0.3497717083,
-0.0467959121,
0.0411746651,
0.0660476238,
-0.2239822894,
-0.1087347493,
0.1983458549,
0.3862471879,
-0.0009005222,
-0.2026980668,
0.1171665862,
-0.3669370115,
0.1662435383,
-0.1405932158,
0.4673705995,
-0.2569172084,
0.1729713529,
-0.0228857659,
-0.3501436412,
-0.3971062303,
-0.1263404489,
-0.2659688294,
-0.113525264,
-0.3912785649,
-0.1805746853,
-0.1014031246,
-0.0171222407,
0.1442001611,
-0.0524011739,
-0.12381199,
-0.270158112,
-0.0278829653,
0.0554123931,
-0.0799710453,
-0.123778604,
0.0732187554,
0.1210664064,
-0.347232312,
-0.0554115362,
0.1460537612,
-0.039532613,
0.226110369,
0.4076478183,
-0.5039839149,
0.0725947544,
0.2214099914,
0.2120588869,
0.3264288902,
0.3990578353,
0.0824618563,
0.4527713656,
0.1557212621,
0.0685369447,
-0.0174625851,
0.0426177047,
-0.2237728685,
-0.0387068987,
0.0853187665,
0.1677064002,
0.1316860616,
0.2200067639,
0.2272941023,
0.1832954586,
-0.1064096242,
0.0482757241,
0.1594004482,
0.3050546348,
-0.2743835449,
0.1071229577,
-0.2487889677,
0.3690177202,
-0.0323476307,
0.177942127,
0.171196118,
0.0399643183,
-0.0335227586,
0.0794005692,
0.0834059045,
0.5254685879,
-0.0163511448,
0.1120553389,
-0.0185537152,
0.4008261859,
0.2523583174,
-0.2061772197,
0.1637970805,
0.0349365324,
0.3331682086,
-0.1194412932,
-0.2856723666,
0.29205513,
0.3565747142,
-0.1682104617,
0.3340027928,
0.183313787,
-0.0667126924,
0.0179810971,
-0.0859552696,
0.1677902639,
-0.0120944167,
-0.0016967515,
-0.7779325247,
0.0512740351,
-0.0561567023,
-0.1049492955,
0.1208094731,
-0.0815202147,
0.1709188521,
0.3347249329,
-0.2347366363,
-0.1270286292,
0.0550201349,
-0.0758107603,
-0.1394141018,
-0.0509181283,
0.1089926958,
0.2049473673,
0.074008368,
-0.2799780667,
0.2123621553,
0.0756097808,
0.1040028706,
-0.1048163921,
0.4057818055,
0.2850219309,
-0.0817154497,
0.0166609976,
-0.0904396549,
0.2010721713,
0.1049899608,
-0.2600869238,
0.0940877944,
0.3385108709,
0.0787671655,
0.2597361803,
0.2011121809,
-0.1343291849,
-0.2416918576,
-0.2874893546,
0.050415013,
-0.1882930398,
0.1537922025,
-0.1491673738,
-0.0916519463,
0.0543052554,
0.1305519193,
-0.4923563898,
0.2276897728,
0.319354713,
0.0556117333,
0.1301304549,
-0.0193795059,
0.1160943732,
-0.2794569433,
-0.0227173194,
0.2474241406,
0.2013017386,
0.0879413262,
-0.5815749764,
-0.0923746526,
0.3105399013,
-0.1654672623,
-0.3448003531,
0.0532618761,
0.3007674217,
0.0372679569,
0.0376977324,
0.1974072754,
-0.1079813763,
-0.2613868117,
0.1192396432,
-0.305313766,
0.0157388356,
0.0029158548,
0.0249561444,
0.1774375737,
-0.0161395725,
0.0565106384,
-0.1310530305,
0.0159717109,
-0.1169695184,
0.0239996836,
0.3244974315,
0.2114357501,
0.3351795077,
0.1502849907,
0.4356199503,
-0.1151319966,
-0.3086130321,
-0.1074877083,
0.0539790541,
0.0432385467,
-0.1012954861,
-0.337330848,
-0.2797783315,
-0.1999593675,
0.0397300199,
0.2189146876,
0.4644092917,
0.2239392847,
-0.1449688226,
-0.2340931296,
-0.0960400999,
-0.1071276069,
-0.3327948749,
0.173146382,
0.256965816,
-0.1455278844,
-0.2706969976,
0.0175239872,
-0.0046457588,
0.3147621155,
-0.181398958,
0.1084276736,
-0.3498394191,
-0.1541063339,
0.2446237206,
-0.1181627512,
-0.4130527377,
0.0719896778,
0.376216501,
0.3241553903,
-0.0967501998,
0.3059367537,
0.1406516582,
-0.188891381,
-0.0053486261,
0.7830972672,
-0.307081461,
-0.4296426177,
-0.1124285012,
-0.0667240471
]
|
https://github.com/huggingface/datasets/issues/1981 | wmt datasets fail to load | yes, of course, I reverted to the version before that and it works ;)
but since a new release was just made you will probably need to make a hotfix.
and add the wmt to the tests? | on master:
```
python -c 'from datasets import load_dataset; load_dataset("wmt14", "de-en")'
Downloading and preparing dataset wmt14/de-en (download: Unknown size, generated: Unknown size, post-processed: Unknown size, total: Unknown size) to /home/stas/.cache/huggingface/datasets/wmt14/de-en/1.0.0/43e717d978d2261502b0194999583acb874ba73b0f4aed0ada2889d1bb00f36e...
Traceback (most recent call last):
File "<string>", line 1, in <module>
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/builder.py", line 578, in download_and_prepare
self._download_and_prepare(
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/builder.py", line 634, in _download_and_prepare
split_generators = self._split_generators(dl_manager, **split_generators_kwargs)
File "/home/stas/.cache/huggingface/modules/datasets_modules/datasets/wmt14/43e717d978d2261502b0194999583acb874ba73b0f4aed0ada2889d1bb00f36e/wmt_utils.py", line 760, in _split_generators
extraction_map = dict(downloaded_files, **manual_files)
```
it worked fine recently. same problem if I try wmt16.
git bisect points to this commit from Feb 25 as the culprit https://github.com/huggingface/datasets/commit/792f1d9bb1c5361908f73e2ef7f0181b2be409fa
@albertvillanova | 37 | wmt datasets fail to load
on master:
```
python -c 'from datasets import load_dataset; load_dataset("wmt14", "de-en")'
Downloading and preparing dataset wmt14/de-en (download: Unknown size, generated: Unknown size, post-processed: Unknown size, total: Unknown size) to /home/stas/.cache/huggingface/datasets/wmt14/de-en/1.0.0/43e717d978d2261502b0194999583acb874ba73b0f4aed0ada2889d1bb00f36e...
Traceback (most recent call last):
File "<string>", line 1, in <module>
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/builder.py", line 578, in download_and_prepare
self._download_and_prepare(
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/builder.py", line 634, in _download_and_prepare
split_generators = self._split_generators(dl_manager, **split_generators_kwargs)
File "/home/stas/.cache/huggingface/modules/datasets_modules/datasets/wmt14/43e717d978d2261502b0194999583acb874ba73b0f4aed0ada2889d1bb00f36e/wmt_utils.py", line 760, in _split_generators
extraction_map = dict(downloaded_files, **manual_files)
```
it worked fine recently. same problem if I try wmt16.
git bisect points to this commit from Feb 25 as the culprit https://github.com/huggingface/datasets/commit/792f1d9bb1c5361908f73e2ef7f0181b2be409fa
@albertvillanova
yes, of course, I reverted to the version before that and it works ;)
but since a new release was just made you will probably need to make a hotfix.
and add the wmt to the tests? | [
-0.3290720284,
-0.0584941655,
-0.0104082497,
0.5395738482,
0.31043154,
0.0041046697,
0.209779799,
0.0872705206,
0.3129353225,
0.1078863814,
-0.0224997792,
-0.1256617457,
-0.296931684,
0.1887944639,
0.1114162803,
0.1848194599,
-0.1364243627,
0.005854344,
-0.8216049671,
0.015868552,
-0.1838497072,
0.1984841824,
-0.1673420817,
-0.0969725102,
-0.5068027377,
0.2375524342,
-0.0300086811,
0.2536422312,
-0.211371094,
-0.4816321731,
0.5141908526,
0.0309753548,
0.0518514104,
0.557684958,
-0.0001141044,
0.1515251994,
0.2935241461,
-0.010245854,
-0.2466025651,
-0.2974116802,
-0.5779014826,
-0.3827317953,
0.0219600908,
0.2245496064,
-0.1884620488,
0.0820961893,
-0.2400668859,
-0.3640689254,
0.282050699,
0.2943235934,
0.2214950323,
0.4151585996,
0.2789464295,
-0.3091941774,
0.0510981493,
0.2907714248,
-0.1379878372,
-0.0280018132,
0.0135749867,
-0.1352171302,
0.0232768413,
0.0316456817,
0.0733231157,
0.329772085,
0.3457941115,
-0.1587232798,
0.2857410312,
-0.0081031621,
0.279225111,
0.0577793084,
0.1964945942,
-0.0757791251,
-0.2360397577,
-0.0594308041,
-0.1306952089,
-0.4276536107,
0.259202987,
0.2658745646,
0.0303439982,
0.2660062611,
-0.3589411378,
0.0739578232,
0.2212081701,
0.0317870416,
-0.3881676197,
0.3771409988,
-0.311494112,
0.0018199214,
0.2749301493,
-0.1902686208,
0.1660658568,
-0.111459583,
-0.0187489502,
0.0837208107,
-0.5072568655,
0.2616915107,
0.0255592316,
0.2756200433,
-0.08005739,
0.1582368016,
-0.252016753,
0.0149275595,
0.2089378387,
-0.0155650964,
0.178793028,
0.1386246383,
-0.1099449173,
-0.062659435,
0.2749223113,
0.0550981089,
-0.185341835,
0.1351207793,
-0.1703787744,
-0.4433654547,
-0.0891755149,
-0.1894307137,
0.1525505632,
-0.0720275491,
-0.259921819,
0.0635319725,
0.1355185807,
-0.3142132163,
0.0204186961,
0.2855194807,
0.0484093018,
0.1702364236,
0.1179612651,
0.0036532658,
-0.0042030206,
-0.0357706435,
-0.3564470708,
-0.3906193972,
-0.2991640568,
0.0611201636,
0.4078392684,
-0.2185732573,
0.2283027619,
0.0004110408,
-0.1310047656,
-0.1273209751,
-0.2269292325,
-0.0077420096,
0.2188935429,
0.4824035764,
0.0710931048,
0.2990787029,
0.2443662882,
0.1595918089,
-0.0081309741,
-0.0074383928,
-0.0995393172,
-0.2956008315,
0.1295209378,
0.2581728995,
-0.0815233514,
0.1570642889,
-0.229450345,
-0.1136633679,
0.1050200686,
0.3215998113,
-0.1928716004,
-0.241754815,
-0.1637250632,
-0.066638723,
0.5321368575,
0.5977165103,
0.0095659606,
-0.1942434162,
-0.3947502375,
-0.0471859053,
0.2344341278,
0.332223475,
-0.0298236217,
0.100702621,
-0.2609954476,
-0.2470606416,
-0.08204595,
-0.059450306,
-0.1832844615,
0.1371453106,
-0.131323874,
0.2751170397,
0.0324516147,
-0.126380235,
0.014531306,
-0.2265357226,
0.34289065,
0.0891365707,
-0.1580775976,
0.0836278349,
-0.0794163123,
-0.3164913952,
0.2474275678,
0.1886272132,
0.1936814338,
-0.1896168292,
0.2157250196,
0.0242878385,
0.06840913,
0.0570892431,
0.1292503327,
0.0385190472,
0.0848580003,
-0.0127782077,
-0.0889183357,
0.0534028187,
-0.4473015964,
0.2964878976,
0.2691924572,
0.0437412411,
0.1173464507,
0.1208165735,
-0.1622186154,
0.1335768253,
-0.3434742987,
-0.1887685508,
0.0876792744,
0.1349027455,
-0.0035154345,
-0.1450344026,
-0.038808696,
-0.0239785258,
-0.2259173095,
0.1487141103,
0.2445328683,
0.0667197108,
-0.1433454603,
-0.1252085865,
0.2802848816,
0.2743448615,
0.1635605544,
-0.1697693765,
-0.1630632281,
0.0908570811,
0.1399754733,
0.395195514,
-0.0871866122,
0.103921324,
0.1791916192,
-0.4736975133,
0.1699436754,
0.1361611038,
-0.1402690262,
-0.2992377877,
0.1204288751,
0.0424619354,
-0.1103156358,
0.2675851285,
0.1175593138,
0.0357345417,
0.1648183167,
0.0709900782,
0.1453140825,
-0.3879216909,
0.2801465392,
-0.0923156142,
0.3428555429,
0.2670228183,
0.0239536855,
-0.0425496697,
0.3805398047,
0.1634020656,
0.202234596,
0.0150983231,
-0.3856003582,
-0.0856610835,
-0.0661872029,
0.0501850769,
0.3967729211,
0.0735272542,
0.0935866609,
0.1786359847,
0.114971444,
-0.3025265634,
0.4467022717,
-0.0749786347,
-0.0767070204,
0.3043872416,
-0.0235596094,
-0.0722773448,
-0.2620559335,
0.5669640899,
0.0582100488,
0.0062174299,
-0.1975156069,
-0.2630081773,
-0.4342719316,
0.2357836813,
-0.3500566185,
-0.5691423416,
-0.2052635103,
-0.205743283,
-0.2758674026,
0.1181361899,
-0.0988536924,
0.2478710711,
0.0687463805,
0.2188891619,
-0.0490785055,
0.3465980291,
-0.0977025628,
-0.2259615213,
-0.1064978391,
0.0391054153,
0.5312061906,
-0.2995473444,
0.3275357783,
-0.1957135946,
0.0639885589,
-0.200259313,
-0.2282970548,
0.0872321948,
-0.0478703156,
0.3465408385,
0.2117683738,
-0.0232821815,
0.3644335568,
-0.1089352816,
0.3259526789,
-0.1572975963,
-0.0153348055,
0.0034697694,
-0.0445442982,
-0.1426704675,
-0.2228536606,
-0.3446231186,
-0.4408052564,
-0.388345331,
-0.0106529063,
-0.0261882301,
-0.0418935753,
0.0917573795,
-0.1342887729,
-0.0532306097,
-0.0178113207,
-0.0258908607,
-0.3486144543,
-0.2984479368,
0.2000668794,
-0.3020641208,
-0.2819244266,
0.239293173,
0.1354365349,
0.4544389248,
0.0401276536,
-0.3937390447,
0.5808199048,
-0.1391111463,
-0.114262931,
0.1804575324,
0.3090637326,
-0.0905660689,
0.1654046625,
-0.0495853201,
-0.1657476574,
-0.2646008134,
-0.1806015223,
-0.2320664823,
0.1591045558,
-0.1456698477,
0.0627861172,
0.0104182102,
0.8047190905,
0.0654787272,
-0.2943532467,
0.1827454716,
0.1267127097,
0.2218525857,
-0.00069613,
-0.5895356536,
0.2010114342,
-0.0986169949,
0.2215836346,
0.3626621962,
0.043290507,
0.1737744957,
-0.1139221862,
0.1939599067,
-0.1887793839,
-0.3513716459,
-0.1281633228,
0.2005106509,
0.3849680722,
0.0900529847,
0.1219887733,
0.0273913853,
-0.0954924673,
-0.0095354188,
0.3547011018,
0.1222475618,
0.0885328799,
-0.1050637513,
-0.0560124964,
-0.2369754314,
0.0535889491,
-0.1932055205,
0.4575622976,
-0.0324501917,
-0.1230475605,
-0.0423156507,
-0.0182905514,
0.5026885867,
0.1967384666,
0.3927700222,
0.1022481918,
-0.4248225689,
-0.2393002212,
-0.1275757402,
0.0902223736,
-0.0735772923,
0.1285208017,
0.1846210957,
-0.2020291835,
-0.5072084069,
0.2025790662,
0.1107910275,
-0.1888127476,
-0.1198270246,
-0.1453296542,
0.0249492191,
-0.2902212739,
-0.0777403265,
-0.2248144597,
0.1797916144,
-0.4049387276,
-0.0239726696,
-0.1545185894,
-0.0788379833,
0.1511587799,
0.065721795,
0.0934650525,
0.0443000235,
0.4923815429,
0.3122970462,
0.3200490773,
0.5650812387,
0.6159528494,
-0.1010638475,
0.0344184749,
0.083505556,
0.0163795929,
0.0522826947,
0.2831605077,
-0.0781764761,
0.111100398,
0.0438613966,
0.1107480004,
-0.596083045,
0.1240011081,
0.2019081116,
0.0680200979,
-0.357242316,
-0.0997594744,
0.1946077496,
0.0268736612,
0.2006979585,
0.176009953,
0.1702609509,
-0.3972955346,
-0.4666158855,
0.0168192126,
0.494731307,
-0.0735876039,
0.2222426087,
-0.1423189491,
-0.1103768498,
0.0566483587,
0.2778767943,
-0.1172654331,
-0.191495344,
0.17908521,
0.031930849,
-0.1585559994,
0.0860941932,
0.1152570173,
-0.0740364119,
0.2761103511,
0.0473109894,
0.5473354459,
0.1399032772,
0.0827617273,
-0.133387953,
-0.2570033967,
-0.2534720302,
0.1469008476,
-0.0401019156,
0.3355661333,
-0.2314385772,
-0.1019252166,
0.3119748831,
-0.0665351152,
-0.2452949286,
0.0065799658,
-0.6495984197,
0.1033414751,
-0.0357613713,
-0.10310179,
0.3692241311,
-0.2313643992,
0.1811164171,
0.3199763894,
-0.1902632564,
0.1010997146,
-0.3137946725,
0.0383314714,
-0.2302721739,
0.2646710277,
0.100801602,
-0.0336171463,
-0.0603651293,
-0.1086392254,
-0.0407439806,
-0.0539292656,
0.0338525698,
0.0507711321,
-0.055291675,
-0.0493356995,
-0.3194901943,
0.0118200779,
0.0131510403,
-0.1511107981,
0.1543609947,
0.0290809143,
-0.0549285747,
0.3291853666,
-0.0549550913,
-0.1067547947,
-0.0585502312,
0.4145899415,
-0.2623611391,
0.0229863096,
0.3038801551,
0.4151067436,
-0.0175479371,
-0.2813272178,
-0.068515338,
0.0908065215,
-0.2448112816,
0.2701679766,
0.1164216697,
-0.0899813846,
0.3801681697,
0.5611447096,
0.3422604203,
-0.1498253495,
0.0122463014,
-0.2868365049,
-0.1911179572,
0.0608149767,
-0.1435581595,
0.1625947207,
0.0277144164,
0.4404928386,
-0.1747052372,
0.3926444352,
-0.3059358597,
0.2749380171,
-0.0947573632,
-0.0000581009,
0.2123727202,
-0.1585533321,
0.1591584831,
-0.0921345279,
0.0412249081,
-0.0340269804,
-0.5342017412,
-0.2137607485,
-0.1178594679,
0.0945380926,
0.0980820432,
-0.2711147368,
0.1690741181,
-0.1080358848,
0.0807956308,
-0.1697109938,
0.3470470011,
0.0660691932,
-0.1222604066,
0.0229023024,
0.0703014061,
0.0658249035,
-0.168961376,
0.1390582174,
0.071453236,
-0.067727305,
-0.0049926243,
-0.0410778932,
0.0441779383,
0.0208380353,
0.1150302738,
0.010286944,
-0.0216587577,
0.1182221621,
0.5094001293,
-0.3056087792,
-0.2525113225,
0.2638110518,
0.2856665254,
0.1686182767,
0.0513614826,
0.0614276715,
-0.1690222174,
0.1712697595,
-0.2953844368,
-0.079539448,
-0.037757352,
0.1637817472,
0.0857126117,
0.0499725528,
0.3570750058,
-0.2151892781,
-0.2337027043,
0.3549879789,
0.3251189888,
-0.1116919667,
0.1748535186,
0.4612628818,
0.0847790688,
0.2086673379,
0.1075343117,
-0.1653203368,
0.1047749072,
0.3765079081,
-0.2396266609,
0.6803705692,
0.0646546334,
0.0063732942,
0.2407554835,
-0.4143281877,
0.1107712686,
0.2690978944,
-0.1568296105,
0.0244204495,
-0.2630804479,
0.1377332807,
-0.340200752,
-0.2731530964,
-0.1855366379,
0.1984927952,
-0.0655510426,
-0.1254136115,
-0.2556760013,
-0.1166857928,
-0.0780742839,
0.0273625441,
-0.0752226338,
-0.102267839,
0.2595923841,
0.1732609868,
-0.1564744711,
-0.4046474993,
-0.3694780767,
0.0161440447,
-0.0983203799,
-0.1228211895,
0.2560080886,
0.4048369229,
-0.2123368531,
0.1384949088,
0.4051624238,
0.4715677798,
0.3013881147,
-0.0192861278,
-0.0860338286,
0.0871974751,
-0.0851399228,
-0.277500242,
0.4197262824,
-0.2568071783,
-0.1573419273,
0.0049491497,
0.1699632853,
-0.1862419844,
-0.3644251525,
0.1278569251,
-0.3126043379,
-0.283562094,
0.1925963014,
-0.0886966735,
-0.057648968,
-0.0464048795,
0.3801314831,
-0.046357248,
0.2271073759,
0.6677178741,
-0.0275355075,
0.1280926317,
-0.2423587143,
0.1004885733,
0.0852619261,
0.7199056745,
0.2930561304,
-0.0817144364,
-0.2865350842,
-0.5099944472,
-0.781591475,
0.0482606329,
0.1542912275,
0.4362872243,
0.0736526847,
0.0439880788,
0.1192256212,
-0.0225300677,
0.1537164599,
0.122018531,
0.2925756872,
-0.2151332051,
-0.3548650742,
0.1796958297,
-0.0950154215,
-0.1906717718,
-0.0053812056,
-0.3220203221,
0.1466881186,
-0.2788924873,
0.0247031543,
-0.2417235225,
0.4235900044,
0.0029750341,
0.2875812948,
0.2188120335,
0.0880906209,
0.306371212,
0.0107986862,
-0.0630574971,
-0.4197324216,
-0.3112782538,
0.0145373214,
0.086073719,
-0.1074680313,
0.3646840751,
-0.0537012331,
-0.2685219049,
-0.4432088733,
0.105085738,
0.1143685207,
0.2023359239,
-0.1769941002,
0.2222106755,
-0.2147683799,
-0.1733143181,
0.3443756104,
0.4282422364,
-0.0292371009,
0.187837109,
-0.2106768787,
-0.3873318434,
0.2546338141,
-0.2887780964,
-0.1629845053,
-0.4629841745,
0.1772363037,
-0.0954382494,
-0.1698632091,
-0.4217801392,
0.152843684,
0.1712171882,
-0.1016815007,
-0.2147470266,
0.3726131022,
-0.0460542291,
-0.0684141368,
-0.0185813867,
0.1694672555,
0.1803848594,
-0.3343188167,
-0.1139622703,
-0.0598334782
]
|
https://github.com/huggingface/datasets/issues/1981 | wmt datasets fail to load | @stas00 it is fixed. @lhoestq are you releasing the hot fix or would you prefer me to do it? | on master:
```
python -c 'from datasets import load_dataset; load_dataset("wmt14", "de-en")'
Downloading and preparing dataset wmt14/de-en (download: Unknown size, generated: Unknown size, post-processed: Unknown size, total: Unknown size) to /home/stas/.cache/huggingface/datasets/wmt14/de-en/1.0.0/43e717d978d2261502b0194999583acb874ba73b0f4aed0ada2889d1bb00f36e...
Traceback (most recent call last):
File "<string>", line 1, in <module>
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/builder.py", line 578, in download_and_prepare
self._download_and_prepare(
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/builder.py", line 634, in _download_and_prepare
split_generators = self._split_generators(dl_manager, **split_generators_kwargs)
File "/home/stas/.cache/huggingface/modules/datasets_modules/datasets/wmt14/43e717d978d2261502b0194999583acb874ba73b0f4aed0ada2889d1bb00f36e/wmt_utils.py", line 760, in _split_generators
extraction_map = dict(downloaded_files, **manual_files)
```
it worked fine recently. same problem if I try wmt16.
git bisect points to this commit from Feb 25 as the culprit https://github.com/huggingface/datasets/commit/792f1d9bb1c5361908f73e2ef7f0181b2be409fa
@albertvillanova | 19 | wmt datasets fail to load
on master:
```
python -c 'from datasets import load_dataset; load_dataset("wmt14", "de-en")'
Downloading and preparing dataset wmt14/de-en (download: Unknown size, generated: Unknown size, post-processed: Unknown size, total: Unknown size) to /home/stas/.cache/huggingface/datasets/wmt14/de-en/1.0.0/43e717d978d2261502b0194999583acb874ba73b0f4aed0ada2889d1bb00f36e...
Traceback (most recent call last):
File "<string>", line 1, in <module>
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/builder.py", line 578, in download_and_prepare
self._download_and_prepare(
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/builder.py", line 634, in _download_and_prepare
split_generators = self._split_generators(dl_manager, **split_generators_kwargs)
File "/home/stas/.cache/huggingface/modules/datasets_modules/datasets/wmt14/43e717d978d2261502b0194999583acb874ba73b0f4aed0ada2889d1bb00f36e/wmt_utils.py", line 760, in _split_generators
extraction_map = dict(downloaded_files, **manual_files)
```
it worked fine recently. same problem if I try wmt16.
git bisect points to this commit from Feb 25 as the culprit https://github.com/huggingface/datasets/commit/792f1d9bb1c5361908f73e2ef7f0181b2be409fa
@albertvillanova
@stas00 it is fixed. @lhoestq are you releasing the hot fix or would you prefer me to do it? | [
-0.3290720284,
-0.0584941655,
-0.0104082497,
0.5395738482,
0.31043154,
0.0041046697,
0.209779799,
0.0872705206,
0.3129353225,
0.1078863814,
-0.0224997792,
-0.1256617457,
-0.296931684,
0.1887944639,
0.1114162803,
0.1848194599,
-0.1364243627,
0.005854344,
-0.8216049671,
0.015868552,
-0.1838497072,
0.1984841824,
-0.1673420817,
-0.0969725102,
-0.5068027377,
0.2375524342,
-0.0300086811,
0.2536422312,
-0.211371094,
-0.4816321731,
0.5141908526,
0.0309753548,
0.0518514104,
0.557684958,
-0.0001141044,
0.1515251994,
0.2935241461,
-0.010245854,
-0.2466025651,
-0.2974116802,
-0.5779014826,
-0.3827317953,
0.0219600908,
0.2245496064,
-0.1884620488,
0.0820961893,
-0.2400668859,
-0.3640689254,
0.282050699,
0.2943235934,
0.2214950323,
0.4151585996,
0.2789464295,
-0.3091941774,
0.0510981493,
0.2907714248,
-0.1379878372,
-0.0280018132,
0.0135749867,
-0.1352171302,
0.0232768413,
0.0316456817,
0.0733231157,
0.329772085,
0.3457941115,
-0.1587232798,
0.2857410312,
-0.0081031621,
0.279225111,
0.0577793084,
0.1964945942,
-0.0757791251,
-0.2360397577,
-0.0594308041,
-0.1306952089,
-0.4276536107,
0.259202987,
0.2658745646,
0.0303439982,
0.2660062611,
-0.3589411378,
0.0739578232,
0.2212081701,
0.0317870416,
-0.3881676197,
0.3771409988,
-0.311494112,
0.0018199214,
0.2749301493,
-0.1902686208,
0.1660658568,
-0.111459583,
-0.0187489502,
0.0837208107,
-0.5072568655,
0.2616915107,
0.0255592316,
0.2756200433,
-0.08005739,
0.1582368016,
-0.252016753,
0.0149275595,
0.2089378387,
-0.0155650964,
0.178793028,
0.1386246383,
-0.1099449173,
-0.062659435,
0.2749223113,
0.0550981089,
-0.185341835,
0.1351207793,
-0.1703787744,
-0.4433654547,
-0.0891755149,
-0.1894307137,
0.1525505632,
-0.0720275491,
-0.259921819,
0.0635319725,
0.1355185807,
-0.3142132163,
0.0204186961,
0.2855194807,
0.0484093018,
0.1702364236,
0.1179612651,
0.0036532658,
-0.0042030206,
-0.0357706435,
-0.3564470708,
-0.3906193972,
-0.2991640568,
0.0611201636,
0.4078392684,
-0.2185732573,
0.2283027619,
0.0004110408,
-0.1310047656,
-0.1273209751,
-0.2269292325,
-0.0077420096,
0.2188935429,
0.4824035764,
0.0710931048,
0.2990787029,
0.2443662882,
0.1595918089,
-0.0081309741,
-0.0074383928,
-0.0995393172,
-0.2956008315,
0.1295209378,
0.2581728995,
-0.0815233514,
0.1570642889,
-0.229450345,
-0.1136633679,
0.1050200686,
0.3215998113,
-0.1928716004,
-0.241754815,
-0.1637250632,
-0.066638723,
0.5321368575,
0.5977165103,
0.0095659606,
-0.1942434162,
-0.3947502375,
-0.0471859053,
0.2344341278,
0.332223475,
-0.0298236217,
0.100702621,
-0.2609954476,
-0.2470606416,
-0.08204595,
-0.059450306,
-0.1832844615,
0.1371453106,
-0.131323874,
0.2751170397,
0.0324516147,
-0.126380235,
0.014531306,
-0.2265357226,
0.34289065,
0.0891365707,
-0.1580775976,
0.0836278349,
-0.0794163123,
-0.3164913952,
0.2474275678,
0.1886272132,
0.1936814338,
-0.1896168292,
0.2157250196,
0.0242878385,
0.06840913,
0.0570892431,
0.1292503327,
0.0385190472,
0.0848580003,
-0.0127782077,
-0.0889183357,
0.0534028187,
-0.4473015964,
0.2964878976,
0.2691924572,
0.0437412411,
0.1173464507,
0.1208165735,
-0.1622186154,
0.1335768253,
-0.3434742987,
-0.1887685508,
0.0876792744,
0.1349027455,
-0.0035154345,
-0.1450344026,
-0.038808696,
-0.0239785258,
-0.2259173095,
0.1487141103,
0.2445328683,
0.0667197108,
-0.1433454603,
-0.1252085865,
0.2802848816,
0.2743448615,
0.1635605544,
-0.1697693765,
-0.1630632281,
0.0908570811,
0.1399754733,
0.395195514,
-0.0871866122,
0.103921324,
0.1791916192,
-0.4736975133,
0.1699436754,
0.1361611038,
-0.1402690262,
-0.2992377877,
0.1204288751,
0.0424619354,
-0.1103156358,
0.2675851285,
0.1175593138,
0.0357345417,
0.1648183167,
0.0709900782,
0.1453140825,
-0.3879216909,
0.2801465392,
-0.0923156142,
0.3428555429,
0.2670228183,
0.0239536855,
-0.0425496697,
0.3805398047,
0.1634020656,
0.202234596,
0.0150983231,
-0.3856003582,
-0.0856610835,
-0.0661872029,
0.0501850769,
0.3967729211,
0.0735272542,
0.0935866609,
0.1786359847,
0.114971444,
-0.3025265634,
0.4467022717,
-0.0749786347,
-0.0767070204,
0.3043872416,
-0.0235596094,
-0.0722773448,
-0.2620559335,
0.5669640899,
0.0582100488,
0.0062174299,
-0.1975156069,
-0.2630081773,
-0.4342719316,
0.2357836813,
-0.3500566185,
-0.5691423416,
-0.2052635103,
-0.205743283,
-0.2758674026,
0.1181361899,
-0.0988536924,
0.2478710711,
0.0687463805,
0.2188891619,
-0.0490785055,
0.3465980291,
-0.0977025628,
-0.2259615213,
-0.1064978391,
0.0391054153,
0.5312061906,
-0.2995473444,
0.3275357783,
-0.1957135946,
0.0639885589,
-0.200259313,
-0.2282970548,
0.0872321948,
-0.0478703156,
0.3465408385,
0.2117683738,
-0.0232821815,
0.3644335568,
-0.1089352816,
0.3259526789,
-0.1572975963,
-0.0153348055,
0.0034697694,
-0.0445442982,
-0.1426704675,
-0.2228536606,
-0.3446231186,
-0.4408052564,
-0.388345331,
-0.0106529063,
-0.0261882301,
-0.0418935753,
0.0917573795,
-0.1342887729,
-0.0532306097,
-0.0178113207,
-0.0258908607,
-0.3486144543,
-0.2984479368,
0.2000668794,
-0.3020641208,
-0.2819244266,
0.239293173,
0.1354365349,
0.4544389248,
0.0401276536,
-0.3937390447,
0.5808199048,
-0.1391111463,
-0.114262931,
0.1804575324,
0.3090637326,
-0.0905660689,
0.1654046625,
-0.0495853201,
-0.1657476574,
-0.2646008134,
-0.1806015223,
-0.2320664823,
0.1591045558,
-0.1456698477,
0.0627861172,
0.0104182102,
0.8047190905,
0.0654787272,
-0.2943532467,
0.1827454716,
0.1267127097,
0.2218525857,
-0.00069613,
-0.5895356536,
0.2010114342,
-0.0986169949,
0.2215836346,
0.3626621962,
0.043290507,
0.1737744957,
-0.1139221862,
0.1939599067,
-0.1887793839,
-0.3513716459,
-0.1281633228,
0.2005106509,
0.3849680722,
0.0900529847,
0.1219887733,
0.0273913853,
-0.0954924673,
-0.0095354188,
0.3547011018,
0.1222475618,
0.0885328799,
-0.1050637513,
-0.0560124964,
-0.2369754314,
0.0535889491,
-0.1932055205,
0.4575622976,
-0.0324501917,
-0.1230475605,
-0.0423156507,
-0.0182905514,
0.5026885867,
0.1967384666,
0.3927700222,
0.1022481918,
-0.4248225689,
-0.2393002212,
-0.1275757402,
0.0902223736,
-0.0735772923,
0.1285208017,
0.1846210957,
-0.2020291835,
-0.5072084069,
0.2025790662,
0.1107910275,
-0.1888127476,
-0.1198270246,
-0.1453296542,
0.0249492191,
-0.2902212739,
-0.0777403265,
-0.2248144597,
0.1797916144,
-0.4049387276,
-0.0239726696,
-0.1545185894,
-0.0788379833,
0.1511587799,
0.065721795,
0.0934650525,
0.0443000235,
0.4923815429,
0.3122970462,
0.3200490773,
0.5650812387,
0.6159528494,
-0.1010638475,
0.0344184749,
0.083505556,
0.0163795929,
0.0522826947,
0.2831605077,
-0.0781764761,
0.111100398,
0.0438613966,
0.1107480004,
-0.596083045,
0.1240011081,
0.2019081116,
0.0680200979,
-0.357242316,
-0.0997594744,
0.1946077496,
0.0268736612,
0.2006979585,
0.176009953,
0.1702609509,
-0.3972955346,
-0.4666158855,
0.0168192126,
0.494731307,
-0.0735876039,
0.2222426087,
-0.1423189491,
-0.1103768498,
0.0566483587,
0.2778767943,
-0.1172654331,
-0.191495344,
0.17908521,
0.031930849,
-0.1585559994,
0.0860941932,
0.1152570173,
-0.0740364119,
0.2761103511,
0.0473109894,
0.5473354459,
0.1399032772,
0.0827617273,
-0.133387953,
-0.2570033967,
-0.2534720302,
0.1469008476,
-0.0401019156,
0.3355661333,
-0.2314385772,
-0.1019252166,
0.3119748831,
-0.0665351152,
-0.2452949286,
0.0065799658,
-0.6495984197,
0.1033414751,
-0.0357613713,
-0.10310179,
0.3692241311,
-0.2313643992,
0.1811164171,
0.3199763894,
-0.1902632564,
0.1010997146,
-0.3137946725,
0.0383314714,
-0.2302721739,
0.2646710277,
0.100801602,
-0.0336171463,
-0.0603651293,
-0.1086392254,
-0.0407439806,
-0.0539292656,
0.0338525698,
0.0507711321,
-0.055291675,
-0.0493356995,
-0.3194901943,
0.0118200779,
0.0131510403,
-0.1511107981,
0.1543609947,
0.0290809143,
-0.0549285747,
0.3291853666,
-0.0549550913,
-0.1067547947,
-0.0585502312,
0.4145899415,
-0.2623611391,
0.0229863096,
0.3038801551,
0.4151067436,
-0.0175479371,
-0.2813272178,
-0.068515338,
0.0908065215,
-0.2448112816,
0.2701679766,
0.1164216697,
-0.0899813846,
0.3801681697,
0.5611447096,
0.3422604203,
-0.1498253495,
0.0122463014,
-0.2868365049,
-0.1911179572,
0.0608149767,
-0.1435581595,
0.1625947207,
0.0277144164,
0.4404928386,
-0.1747052372,
0.3926444352,
-0.3059358597,
0.2749380171,
-0.0947573632,
-0.0000581009,
0.2123727202,
-0.1585533321,
0.1591584831,
-0.0921345279,
0.0412249081,
-0.0340269804,
-0.5342017412,
-0.2137607485,
-0.1178594679,
0.0945380926,
0.0980820432,
-0.2711147368,
0.1690741181,
-0.1080358848,
0.0807956308,
-0.1697109938,
0.3470470011,
0.0660691932,
-0.1222604066,
0.0229023024,
0.0703014061,
0.0658249035,
-0.168961376,
0.1390582174,
0.071453236,
-0.067727305,
-0.0049926243,
-0.0410778932,
0.0441779383,
0.0208380353,
0.1150302738,
0.010286944,
-0.0216587577,
0.1182221621,
0.5094001293,
-0.3056087792,
-0.2525113225,
0.2638110518,
0.2856665254,
0.1686182767,
0.0513614826,
0.0614276715,
-0.1690222174,
0.1712697595,
-0.2953844368,
-0.079539448,
-0.037757352,
0.1637817472,
0.0857126117,
0.0499725528,
0.3570750058,
-0.2151892781,
-0.2337027043,
0.3549879789,
0.3251189888,
-0.1116919667,
0.1748535186,
0.4612628818,
0.0847790688,
0.2086673379,
0.1075343117,
-0.1653203368,
0.1047749072,
0.3765079081,
-0.2396266609,
0.6803705692,
0.0646546334,
0.0063732942,
0.2407554835,
-0.4143281877,
0.1107712686,
0.2690978944,
-0.1568296105,
0.0244204495,
-0.2630804479,
0.1377332807,
-0.340200752,
-0.2731530964,
-0.1855366379,
0.1984927952,
-0.0655510426,
-0.1254136115,
-0.2556760013,
-0.1166857928,
-0.0780742839,
0.0273625441,
-0.0752226338,
-0.102267839,
0.2595923841,
0.1732609868,
-0.1564744711,
-0.4046474993,
-0.3694780767,
0.0161440447,
-0.0983203799,
-0.1228211895,
0.2560080886,
0.4048369229,
-0.2123368531,
0.1384949088,
0.4051624238,
0.4715677798,
0.3013881147,
-0.0192861278,
-0.0860338286,
0.0871974751,
-0.0851399228,
-0.277500242,
0.4197262824,
-0.2568071783,
-0.1573419273,
0.0049491497,
0.1699632853,
-0.1862419844,
-0.3644251525,
0.1278569251,
-0.3126043379,
-0.283562094,
0.1925963014,
-0.0886966735,
-0.057648968,
-0.0464048795,
0.3801314831,
-0.046357248,
0.2271073759,
0.6677178741,
-0.0275355075,
0.1280926317,
-0.2423587143,
0.1004885733,
0.0852619261,
0.7199056745,
0.2930561304,
-0.0817144364,
-0.2865350842,
-0.5099944472,
-0.781591475,
0.0482606329,
0.1542912275,
0.4362872243,
0.0736526847,
0.0439880788,
0.1192256212,
-0.0225300677,
0.1537164599,
0.122018531,
0.2925756872,
-0.2151332051,
-0.3548650742,
0.1796958297,
-0.0950154215,
-0.1906717718,
-0.0053812056,
-0.3220203221,
0.1466881186,
-0.2788924873,
0.0247031543,
-0.2417235225,
0.4235900044,
0.0029750341,
0.2875812948,
0.2188120335,
0.0880906209,
0.306371212,
0.0107986862,
-0.0630574971,
-0.4197324216,
-0.3112782538,
0.0145373214,
0.086073719,
-0.1074680313,
0.3646840751,
-0.0537012331,
-0.2685219049,
-0.4432088733,
0.105085738,
0.1143685207,
0.2023359239,
-0.1769941002,
0.2222106755,
-0.2147683799,
-0.1733143181,
0.3443756104,
0.4282422364,
-0.0292371009,
0.187837109,
-0.2106768787,
-0.3873318434,
0.2546338141,
-0.2887780964,
-0.1629845053,
-0.4629841745,
0.1772363037,
-0.0954382494,
-0.1698632091,
-0.4217801392,
0.152843684,
0.1712171882,
-0.1016815007,
-0.2147470266,
0.3726131022,
-0.0460542291,
-0.0684141368,
-0.0185813867,
0.1694672555,
0.1803848594,
-0.3343188167,
-0.1139622703,
-0.0598334782
]
|
https://github.com/huggingface/datasets/issues/1981 | wmt datasets fail to load | I'll do a patch release for this issue early tomorrow.
And yes we absolutly need tests for the wmt datasets: The missing tests for wmt are an artifact from the early development of the lib but now we have tools to generate automatically the dummy data used for tests :) | on master:
```
python -c 'from datasets import load_dataset; load_dataset("wmt14", "de-en")'
Downloading and preparing dataset wmt14/de-en (download: Unknown size, generated: Unknown size, post-processed: Unknown size, total: Unknown size) to /home/stas/.cache/huggingface/datasets/wmt14/de-en/1.0.0/43e717d978d2261502b0194999583acb874ba73b0f4aed0ada2889d1bb00f36e...
Traceback (most recent call last):
File "<string>", line 1, in <module>
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/builder.py", line 578, in download_and_prepare
self._download_and_prepare(
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/builder.py", line 634, in _download_and_prepare
split_generators = self._split_generators(dl_manager, **split_generators_kwargs)
File "/home/stas/.cache/huggingface/modules/datasets_modules/datasets/wmt14/43e717d978d2261502b0194999583acb874ba73b0f4aed0ada2889d1bb00f36e/wmt_utils.py", line 760, in _split_generators
extraction_map = dict(downloaded_files, **manual_files)
```
it worked fine recently. same problem if I try wmt16.
git bisect points to this commit from Feb 25 as the culprit https://github.com/huggingface/datasets/commit/792f1d9bb1c5361908f73e2ef7f0181b2be409fa
@albertvillanova | 50 | wmt datasets fail to load
on master:
```
python -c 'from datasets import load_dataset; load_dataset("wmt14", "de-en")'
Downloading and preparing dataset wmt14/de-en (download: Unknown size, generated: Unknown size, post-processed: Unknown size, total: Unknown size) to /home/stas/.cache/huggingface/datasets/wmt14/de-en/1.0.0/43e717d978d2261502b0194999583acb874ba73b0f4aed0ada2889d1bb00f36e...
Traceback (most recent call last):
File "<string>", line 1, in <module>
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/builder.py", line 578, in download_and_prepare
self._download_and_prepare(
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/builder.py", line 634, in _download_and_prepare
split_generators = self._split_generators(dl_manager, **split_generators_kwargs)
File "/home/stas/.cache/huggingface/modules/datasets_modules/datasets/wmt14/43e717d978d2261502b0194999583acb874ba73b0f4aed0ada2889d1bb00f36e/wmt_utils.py", line 760, in _split_generators
extraction_map = dict(downloaded_files, **manual_files)
```
it worked fine recently. same problem if I try wmt16.
git bisect points to this commit from Feb 25 as the culprit https://github.com/huggingface/datasets/commit/792f1d9bb1c5361908f73e2ef7f0181b2be409fa
@albertvillanova
I'll do a patch release for this issue early tomorrow.
And yes we absolutly need tests for the wmt datasets: The missing tests for wmt are an artifact from the early development of the lib but now we have tools to generate automatically the dummy data used for tests :) | [
-0.3290720284,
-0.0584941655,
-0.0104082497,
0.5395738482,
0.31043154,
0.0041046697,
0.209779799,
0.0872705206,
0.3129353225,
0.1078863814,
-0.0224997792,
-0.1256617457,
-0.296931684,
0.1887944639,
0.1114162803,
0.1848194599,
-0.1364243627,
0.005854344,
-0.8216049671,
0.015868552,
-0.1838497072,
0.1984841824,
-0.1673420817,
-0.0969725102,
-0.5068027377,
0.2375524342,
-0.0300086811,
0.2536422312,
-0.211371094,
-0.4816321731,
0.5141908526,
0.0309753548,
0.0518514104,
0.557684958,
-0.0001141044,
0.1515251994,
0.2935241461,
-0.010245854,
-0.2466025651,
-0.2974116802,
-0.5779014826,
-0.3827317953,
0.0219600908,
0.2245496064,
-0.1884620488,
0.0820961893,
-0.2400668859,
-0.3640689254,
0.282050699,
0.2943235934,
0.2214950323,
0.4151585996,
0.2789464295,
-0.3091941774,
0.0510981493,
0.2907714248,
-0.1379878372,
-0.0280018132,
0.0135749867,
-0.1352171302,
0.0232768413,
0.0316456817,
0.0733231157,
0.329772085,
0.3457941115,
-0.1587232798,
0.2857410312,
-0.0081031621,
0.279225111,
0.0577793084,
0.1964945942,
-0.0757791251,
-0.2360397577,
-0.0594308041,
-0.1306952089,
-0.4276536107,
0.259202987,
0.2658745646,
0.0303439982,
0.2660062611,
-0.3589411378,
0.0739578232,
0.2212081701,
0.0317870416,
-0.3881676197,
0.3771409988,
-0.311494112,
0.0018199214,
0.2749301493,
-0.1902686208,
0.1660658568,
-0.111459583,
-0.0187489502,
0.0837208107,
-0.5072568655,
0.2616915107,
0.0255592316,
0.2756200433,
-0.08005739,
0.1582368016,
-0.252016753,
0.0149275595,
0.2089378387,
-0.0155650964,
0.178793028,
0.1386246383,
-0.1099449173,
-0.062659435,
0.2749223113,
0.0550981089,
-0.185341835,
0.1351207793,
-0.1703787744,
-0.4433654547,
-0.0891755149,
-0.1894307137,
0.1525505632,
-0.0720275491,
-0.259921819,
0.0635319725,
0.1355185807,
-0.3142132163,
0.0204186961,
0.2855194807,
0.0484093018,
0.1702364236,
0.1179612651,
0.0036532658,
-0.0042030206,
-0.0357706435,
-0.3564470708,
-0.3906193972,
-0.2991640568,
0.0611201636,
0.4078392684,
-0.2185732573,
0.2283027619,
0.0004110408,
-0.1310047656,
-0.1273209751,
-0.2269292325,
-0.0077420096,
0.2188935429,
0.4824035764,
0.0710931048,
0.2990787029,
0.2443662882,
0.1595918089,
-0.0081309741,
-0.0074383928,
-0.0995393172,
-0.2956008315,
0.1295209378,
0.2581728995,
-0.0815233514,
0.1570642889,
-0.229450345,
-0.1136633679,
0.1050200686,
0.3215998113,
-0.1928716004,
-0.241754815,
-0.1637250632,
-0.066638723,
0.5321368575,
0.5977165103,
0.0095659606,
-0.1942434162,
-0.3947502375,
-0.0471859053,
0.2344341278,
0.332223475,
-0.0298236217,
0.100702621,
-0.2609954476,
-0.2470606416,
-0.08204595,
-0.059450306,
-0.1832844615,
0.1371453106,
-0.131323874,
0.2751170397,
0.0324516147,
-0.126380235,
0.014531306,
-0.2265357226,
0.34289065,
0.0891365707,
-0.1580775976,
0.0836278349,
-0.0794163123,
-0.3164913952,
0.2474275678,
0.1886272132,
0.1936814338,
-0.1896168292,
0.2157250196,
0.0242878385,
0.06840913,
0.0570892431,
0.1292503327,
0.0385190472,
0.0848580003,
-0.0127782077,
-0.0889183357,
0.0534028187,
-0.4473015964,
0.2964878976,
0.2691924572,
0.0437412411,
0.1173464507,
0.1208165735,
-0.1622186154,
0.1335768253,
-0.3434742987,
-0.1887685508,
0.0876792744,
0.1349027455,
-0.0035154345,
-0.1450344026,
-0.038808696,
-0.0239785258,
-0.2259173095,
0.1487141103,
0.2445328683,
0.0667197108,
-0.1433454603,
-0.1252085865,
0.2802848816,
0.2743448615,
0.1635605544,
-0.1697693765,
-0.1630632281,
0.0908570811,
0.1399754733,
0.395195514,
-0.0871866122,
0.103921324,
0.1791916192,
-0.4736975133,
0.1699436754,
0.1361611038,
-0.1402690262,
-0.2992377877,
0.1204288751,
0.0424619354,
-0.1103156358,
0.2675851285,
0.1175593138,
0.0357345417,
0.1648183167,
0.0709900782,
0.1453140825,
-0.3879216909,
0.2801465392,
-0.0923156142,
0.3428555429,
0.2670228183,
0.0239536855,
-0.0425496697,
0.3805398047,
0.1634020656,
0.202234596,
0.0150983231,
-0.3856003582,
-0.0856610835,
-0.0661872029,
0.0501850769,
0.3967729211,
0.0735272542,
0.0935866609,
0.1786359847,
0.114971444,
-0.3025265634,
0.4467022717,
-0.0749786347,
-0.0767070204,
0.3043872416,
-0.0235596094,
-0.0722773448,
-0.2620559335,
0.5669640899,
0.0582100488,
0.0062174299,
-0.1975156069,
-0.2630081773,
-0.4342719316,
0.2357836813,
-0.3500566185,
-0.5691423416,
-0.2052635103,
-0.205743283,
-0.2758674026,
0.1181361899,
-0.0988536924,
0.2478710711,
0.0687463805,
0.2188891619,
-0.0490785055,
0.3465980291,
-0.0977025628,
-0.2259615213,
-0.1064978391,
0.0391054153,
0.5312061906,
-0.2995473444,
0.3275357783,
-0.1957135946,
0.0639885589,
-0.200259313,
-0.2282970548,
0.0872321948,
-0.0478703156,
0.3465408385,
0.2117683738,
-0.0232821815,
0.3644335568,
-0.1089352816,
0.3259526789,
-0.1572975963,
-0.0153348055,
0.0034697694,
-0.0445442982,
-0.1426704675,
-0.2228536606,
-0.3446231186,
-0.4408052564,
-0.388345331,
-0.0106529063,
-0.0261882301,
-0.0418935753,
0.0917573795,
-0.1342887729,
-0.0532306097,
-0.0178113207,
-0.0258908607,
-0.3486144543,
-0.2984479368,
0.2000668794,
-0.3020641208,
-0.2819244266,
0.239293173,
0.1354365349,
0.4544389248,
0.0401276536,
-0.3937390447,
0.5808199048,
-0.1391111463,
-0.114262931,
0.1804575324,
0.3090637326,
-0.0905660689,
0.1654046625,
-0.0495853201,
-0.1657476574,
-0.2646008134,
-0.1806015223,
-0.2320664823,
0.1591045558,
-0.1456698477,
0.0627861172,
0.0104182102,
0.8047190905,
0.0654787272,
-0.2943532467,
0.1827454716,
0.1267127097,
0.2218525857,
-0.00069613,
-0.5895356536,
0.2010114342,
-0.0986169949,
0.2215836346,
0.3626621962,
0.043290507,
0.1737744957,
-0.1139221862,
0.1939599067,
-0.1887793839,
-0.3513716459,
-0.1281633228,
0.2005106509,
0.3849680722,
0.0900529847,
0.1219887733,
0.0273913853,
-0.0954924673,
-0.0095354188,
0.3547011018,
0.1222475618,
0.0885328799,
-0.1050637513,
-0.0560124964,
-0.2369754314,
0.0535889491,
-0.1932055205,
0.4575622976,
-0.0324501917,
-0.1230475605,
-0.0423156507,
-0.0182905514,
0.5026885867,
0.1967384666,
0.3927700222,
0.1022481918,
-0.4248225689,
-0.2393002212,
-0.1275757402,
0.0902223736,
-0.0735772923,
0.1285208017,
0.1846210957,
-0.2020291835,
-0.5072084069,
0.2025790662,
0.1107910275,
-0.1888127476,
-0.1198270246,
-0.1453296542,
0.0249492191,
-0.2902212739,
-0.0777403265,
-0.2248144597,
0.1797916144,
-0.4049387276,
-0.0239726696,
-0.1545185894,
-0.0788379833,
0.1511587799,
0.065721795,
0.0934650525,
0.0443000235,
0.4923815429,
0.3122970462,
0.3200490773,
0.5650812387,
0.6159528494,
-0.1010638475,
0.0344184749,
0.083505556,
0.0163795929,
0.0522826947,
0.2831605077,
-0.0781764761,
0.111100398,
0.0438613966,
0.1107480004,
-0.596083045,
0.1240011081,
0.2019081116,
0.0680200979,
-0.357242316,
-0.0997594744,
0.1946077496,
0.0268736612,
0.2006979585,
0.176009953,
0.1702609509,
-0.3972955346,
-0.4666158855,
0.0168192126,
0.494731307,
-0.0735876039,
0.2222426087,
-0.1423189491,
-0.1103768498,
0.0566483587,
0.2778767943,
-0.1172654331,
-0.191495344,
0.17908521,
0.031930849,
-0.1585559994,
0.0860941932,
0.1152570173,
-0.0740364119,
0.2761103511,
0.0473109894,
0.5473354459,
0.1399032772,
0.0827617273,
-0.133387953,
-0.2570033967,
-0.2534720302,
0.1469008476,
-0.0401019156,
0.3355661333,
-0.2314385772,
-0.1019252166,
0.3119748831,
-0.0665351152,
-0.2452949286,
0.0065799658,
-0.6495984197,
0.1033414751,
-0.0357613713,
-0.10310179,
0.3692241311,
-0.2313643992,
0.1811164171,
0.3199763894,
-0.1902632564,
0.1010997146,
-0.3137946725,
0.0383314714,
-0.2302721739,
0.2646710277,
0.100801602,
-0.0336171463,
-0.0603651293,
-0.1086392254,
-0.0407439806,
-0.0539292656,
0.0338525698,
0.0507711321,
-0.055291675,
-0.0493356995,
-0.3194901943,
0.0118200779,
0.0131510403,
-0.1511107981,
0.1543609947,
0.0290809143,
-0.0549285747,
0.3291853666,
-0.0549550913,
-0.1067547947,
-0.0585502312,
0.4145899415,
-0.2623611391,
0.0229863096,
0.3038801551,
0.4151067436,
-0.0175479371,
-0.2813272178,
-0.068515338,
0.0908065215,
-0.2448112816,
0.2701679766,
0.1164216697,
-0.0899813846,
0.3801681697,
0.5611447096,
0.3422604203,
-0.1498253495,
0.0122463014,
-0.2868365049,
-0.1911179572,
0.0608149767,
-0.1435581595,
0.1625947207,
0.0277144164,
0.4404928386,
-0.1747052372,
0.3926444352,
-0.3059358597,
0.2749380171,
-0.0947573632,
-0.0000581009,
0.2123727202,
-0.1585533321,
0.1591584831,
-0.0921345279,
0.0412249081,
-0.0340269804,
-0.5342017412,
-0.2137607485,
-0.1178594679,
0.0945380926,
0.0980820432,
-0.2711147368,
0.1690741181,
-0.1080358848,
0.0807956308,
-0.1697109938,
0.3470470011,
0.0660691932,
-0.1222604066,
0.0229023024,
0.0703014061,
0.0658249035,
-0.168961376,
0.1390582174,
0.071453236,
-0.067727305,
-0.0049926243,
-0.0410778932,
0.0441779383,
0.0208380353,
0.1150302738,
0.010286944,
-0.0216587577,
0.1182221621,
0.5094001293,
-0.3056087792,
-0.2525113225,
0.2638110518,
0.2856665254,
0.1686182767,
0.0513614826,
0.0614276715,
-0.1690222174,
0.1712697595,
-0.2953844368,
-0.079539448,
-0.037757352,
0.1637817472,
0.0857126117,
0.0499725528,
0.3570750058,
-0.2151892781,
-0.2337027043,
0.3549879789,
0.3251189888,
-0.1116919667,
0.1748535186,
0.4612628818,
0.0847790688,
0.2086673379,
0.1075343117,
-0.1653203368,
0.1047749072,
0.3765079081,
-0.2396266609,
0.6803705692,
0.0646546334,
0.0063732942,
0.2407554835,
-0.4143281877,
0.1107712686,
0.2690978944,
-0.1568296105,
0.0244204495,
-0.2630804479,
0.1377332807,
-0.340200752,
-0.2731530964,
-0.1855366379,
0.1984927952,
-0.0655510426,
-0.1254136115,
-0.2556760013,
-0.1166857928,
-0.0780742839,
0.0273625441,
-0.0752226338,
-0.102267839,
0.2595923841,
0.1732609868,
-0.1564744711,
-0.4046474993,
-0.3694780767,
0.0161440447,
-0.0983203799,
-0.1228211895,
0.2560080886,
0.4048369229,
-0.2123368531,
0.1384949088,
0.4051624238,
0.4715677798,
0.3013881147,
-0.0192861278,
-0.0860338286,
0.0871974751,
-0.0851399228,
-0.277500242,
0.4197262824,
-0.2568071783,
-0.1573419273,
0.0049491497,
0.1699632853,
-0.1862419844,
-0.3644251525,
0.1278569251,
-0.3126043379,
-0.283562094,
0.1925963014,
-0.0886966735,
-0.057648968,
-0.0464048795,
0.3801314831,
-0.046357248,
0.2271073759,
0.6677178741,
-0.0275355075,
0.1280926317,
-0.2423587143,
0.1004885733,
0.0852619261,
0.7199056745,
0.2930561304,
-0.0817144364,
-0.2865350842,
-0.5099944472,
-0.781591475,
0.0482606329,
0.1542912275,
0.4362872243,
0.0736526847,
0.0439880788,
0.1192256212,
-0.0225300677,
0.1537164599,
0.122018531,
0.2925756872,
-0.2151332051,
-0.3548650742,
0.1796958297,
-0.0950154215,
-0.1906717718,
-0.0053812056,
-0.3220203221,
0.1466881186,
-0.2788924873,
0.0247031543,
-0.2417235225,
0.4235900044,
0.0029750341,
0.2875812948,
0.2188120335,
0.0880906209,
0.306371212,
0.0107986862,
-0.0630574971,
-0.4197324216,
-0.3112782538,
0.0145373214,
0.086073719,
-0.1074680313,
0.3646840751,
-0.0537012331,
-0.2685219049,
-0.4432088733,
0.105085738,
0.1143685207,
0.2023359239,
-0.1769941002,
0.2222106755,
-0.2147683799,
-0.1733143181,
0.3443756104,
0.4282422364,
-0.0292371009,
0.187837109,
-0.2106768787,
-0.3873318434,
0.2546338141,
-0.2887780964,
-0.1629845053,
-0.4629841745,
0.1772363037,
-0.0954382494,
-0.1698632091,
-0.4217801392,
0.152843684,
0.1712171882,
-0.1016815007,
-0.2147470266,
0.3726131022,
-0.0460542291,
-0.0684141368,
-0.0185813867,
0.1694672555,
0.1803848594,
-0.3343188167,
-0.1139622703,
-0.0598334782
]
|
https://github.com/huggingface/datasets/issues/1981 | wmt datasets fail to load | still facing the same issue or similar:
from datasets import load_dataset
wtm14_test = load_dataset('wmt14',"de-en",cache_dir='./datasets')
~.cache\huggingface\modules\datasets_modules\datasets\wmt14\43e717d978d2261502b0194999583acb874ba73b0f4aed0ada2889d1bb00f36e\wmt_utils.py in _split_generators(self, dl_manager)
758 # Extract manually downloaded files.
759 manual_files = dl_manager.extract(manual_paths_dict)
--> 760 extraction_map = dict(downloaded_files, **manual_files)
761
762 for language in self.config.language_pair:
TypeError: type object argument after ** must be a mapping, not list | on master:
```
python -c 'from datasets import load_dataset; load_dataset("wmt14", "de-en")'
Downloading and preparing dataset wmt14/de-en (download: Unknown size, generated: Unknown size, post-processed: Unknown size, total: Unknown size) to /home/stas/.cache/huggingface/datasets/wmt14/de-en/1.0.0/43e717d978d2261502b0194999583acb874ba73b0f4aed0ada2889d1bb00f36e...
Traceback (most recent call last):
File "<string>", line 1, in <module>
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/builder.py", line 578, in download_and_prepare
self._download_and_prepare(
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/builder.py", line 634, in _download_and_prepare
split_generators = self._split_generators(dl_manager, **split_generators_kwargs)
File "/home/stas/.cache/huggingface/modules/datasets_modules/datasets/wmt14/43e717d978d2261502b0194999583acb874ba73b0f4aed0ada2889d1bb00f36e/wmt_utils.py", line 760, in _split_generators
extraction_map = dict(downloaded_files, **manual_files)
```
it worked fine recently. same problem if I try wmt16.
git bisect points to this commit from Feb 25 as the culprit https://github.com/huggingface/datasets/commit/792f1d9bb1c5361908f73e2ef7f0181b2be409fa
@albertvillanova | 52 | wmt datasets fail to load
on master:
```
python -c 'from datasets import load_dataset; load_dataset("wmt14", "de-en")'
Downloading and preparing dataset wmt14/de-en (download: Unknown size, generated: Unknown size, post-processed: Unknown size, total: Unknown size) to /home/stas/.cache/huggingface/datasets/wmt14/de-en/1.0.0/43e717d978d2261502b0194999583acb874ba73b0f4aed0ada2889d1bb00f36e...
Traceback (most recent call last):
File "<string>", line 1, in <module>
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/load.py", line 740, in load_dataset
builder_instance.download_and_prepare(
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/builder.py", line 578, in download_and_prepare
self._download_and_prepare(
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/builder.py", line 634, in _download_and_prepare
split_generators = self._split_generators(dl_manager, **split_generators_kwargs)
File "/home/stas/.cache/huggingface/modules/datasets_modules/datasets/wmt14/43e717d978d2261502b0194999583acb874ba73b0f4aed0ada2889d1bb00f36e/wmt_utils.py", line 760, in _split_generators
extraction_map = dict(downloaded_files, **manual_files)
```
it worked fine recently. same problem if I try wmt16.
git bisect points to this commit from Feb 25 as the culprit https://github.com/huggingface/datasets/commit/792f1d9bb1c5361908f73e2ef7f0181b2be409fa
@albertvillanova
still facing the same issue or similar:
from datasets import load_dataset
wtm14_test = load_dataset('wmt14',"de-en",cache_dir='./datasets')
~.cache\huggingface\modules\datasets_modules\datasets\wmt14\43e717d978d2261502b0194999583acb874ba73b0f4aed0ada2889d1bb00f36e\wmt_utils.py in _split_generators(self, dl_manager)
758 # Extract manually downloaded files.
759 manual_files = dl_manager.extract(manual_paths_dict)
--> 760 extraction_map = dict(downloaded_files, **manual_files)
761
762 for language in self.config.language_pair:
TypeError: type object argument after ** must be a mapping, not list | [
-0.3290720284,
-0.0584941655,
-0.0104082497,
0.5395738482,
0.31043154,
0.0041046697,
0.209779799,
0.0872705206,
0.3129353225,
0.1078863814,
-0.0224997792,
-0.1256617457,
-0.296931684,
0.1887944639,
0.1114162803,
0.1848194599,
-0.1364243627,
0.005854344,
-0.8216049671,
0.015868552,
-0.1838497072,
0.1984841824,
-0.1673420817,
-0.0969725102,
-0.5068027377,
0.2375524342,
-0.0300086811,
0.2536422312,
-0.211371094,
-0.4816321731,
0.5141908526,
0.0309753548,
0.0518514104,
0.557684958,
-0.0001141044,
0.1515251994,
0.2935241461,
-0.010245854,
-0.2466025651,
-0.2974116802,
-0.5779014826,
-0.3827317953,
0.0219600908,
0.2245496064,
-0.1884620488,
0.0820961893,
-0.2400668859,
-0.3640689254,
0.282050699,
0.2943235934,
0.2214950323,
0.4151585996,
0.2789464295,
-0.3091941774,
0.0510981493,
0.2907714248,
-0.1379878372,
-0.0280018132,
0.0135749867,
-0.1352171302,
0.0232768413,
0.0316456817,
0.0733231157,
0.329772085,
0.3457941115,
-0.1587232798,
0.2857410312,
-0.0081031621,
0.279225111,
0.0577793084,
0.1964945942,
-0.0757791251,
-0.2360397577,
-0.0594308041,
-0.1306952089,
-0.4276536107,
0.259202987,
0.2658745646,
0.0303439982,
0.2660062611,
-0.3589411378,
0.0739578232,
0.2212081701,
0.0317870416,
-0.3881676197,
0.3771409988,
-0.311494112,
0.0018199214,
0.2749301493,
-0.1902686208,
0.1660658568,
-0.111459583,
-0.0187489502,
0.0837208107,
-0.5072568655,
0.2616915107,
0.0255592316,
0.2756200433,
-0.08005739,
0.1582368016,
-0.252016753,
0.0149275595,
0.2089378387,
-0.0155650964,
0.178793028,
0.1386246383,
-0.1099449173,
-0.062659435,
0.2749223113,
0.0550981089,
-0.185341835,
0.1351207793,
-0.1703787744,
-0.4433654547,
-0.0891755149,
-0.1894307137,
0.1525505632,
-0.0720275491,
-0.259921819,
0.0635319725,
0.1355185807,
-0.3142132163,
0.0204186961,
0.2855194807,
0.0484093018,
0.1702364236,
0.1179612651,
0.0036532658,
-0.0042030206,
-0.0357706435,
-0.3564470708,
-0.3906193972,
-0.2991640568,
0.0611201636,
0.4078392684,
-0.2185732573,
0.2283027619,
0.0004110408,
-0.1310047656,
-0.1273209751,
-0.2269292325,
-0.0077420096,
0.2188935429,
0.4824035764,
0.0710931048,
0.2990787029,
0.2443662882,
0.1595918089,
-0.0081309741,
-0.0074383928,
-0.0995393172,
-0.2956008315,
0.1295209378,
0.2581728995,
-0.0815233514,
0.1570642889,
-0.229450345,
-0.1136633679,
0.1050200686,
0.3215998113,
-0.1928716004,
-0.241754815,
-0.1637250632,
-0.066638723,
0.5321368575,
0.5977165103,
0.0095659606,
-0.1942434162,
-0.3947502375,
-0.0471859053,
0.2344341278,
0.332223475,
-0.0298236217,
0.100702621,
-0.2609954476,
-0.2470606416,
-0.08204595,
-0.059450306,
-0.1832844615,
0.1371453106,
-0.131323874,
0.2751170397,
0.0324516147,
-0.126380235,
0.014531306,
-0.2265357226,
0.34289065,
0.0891365707,
-0.1580775976,
0.0836278349,
-0.0794163123,
-0.3164913952,
0.2474275678,
0.1886272132,
0.1936814338,
-0.1896168292,
0.2157250196,
0.0242878385,
0.06840913,
0.0570892431,
0.1292503327,
0.0385190472,
0.0848580003,
-0.0127782077,
-0.0889183357,
0.0534028187,
-0.4473015964,
0.2964878976,
0.2691924572,
0.0437412411,
0.1173464507,
0.1208165735,
-0.1622186154,
0.1335768253,
-0.3434742987,
-0.1887685508,
0.0876792744,
0.1349027455,
-0.0035154345,
-0.1450344026,
-0.038808696,
-0.0239785258,
-0.2259173095,
0.1487141103,
0.2445328683,
0.0667197108,
-0.1433454603,
-0.1252085865,
0.2802848816,
0.2743448615,
0.1635605544,
-0.1697693765,
-0.1630632281,
0.0908570811,
0.1399754733,
0.395195514,
-0.0871866122,
0.103921324,
0.1791916192,
-0.4736975133,
0.1699436754,
0.1361611038,
-0.1402690262,
-0.2992377877,
0.1204288751,
0.0424619354,
-0.1103156358,
0.2675851285,
0.1175593138,
0.0357345417,
0.1648183167,
0.0709900782,
0.1453140825,
-0.3879216909,
0.2801465392,
-0.0923156142,
0.3428555429,
0.2670228183,
0.0239536855,
-0.0425496697,
0.3805398047,
0.1634020656,
0.202234596,
0.0150983231,
-0.3856003582,
-0.0856610835,
-0.0661872029,
0.0501850769,
0.3967729211,
0.0735272542,
0.0935866609,
0.1786359847,
0.114971444,
-0.3025265634,
0.4467022717,
-0.0749786347,
-0.0767070204,
0.3043872416,
-0.0235596094,
-0.0722773448,
-0.2620559335,
0.5669640899,
0.0582100488,
0.0062174299,
-0.1975156069,
-0.2630081773,
-0.4342719316,
0.2357836813,
-0.3500566185,
-0.5691423416,
-0.2052635103,
-0.205743283,
-0.2758674026,
0.1181361899,
-0.0988536924,
0.2478710711,
0.0687463805,
0.2188891619,
-0.0490785055,
0.3465980291,
-0.0977025628,
-0.2259615213,
-0.1064978391,
0.0391054153,
0.5312061906,
-0.2995473444,
0.3275357783,
-0.1957135946,
0.0639885589,
-0.200259313,
-0.2282970548,
0.0872321948,
-0.0478703156,
0.3465408385,
0.2117683738,
-0.0232821815,
0.3644335568,
-0.1089352816,
0.3259526789,
-0.1572975963,
-0.0153348055,
0.0034697694,
-0.0445442982,
-0.1426704675,
-0.2228536606,
-0.3446231186,
-0.4408052564,
-0.388345331,
-0.0106529063,
-0.0261882301,
-0.0418935753,
0.0917573795,
-0.1342887729,
-0.0532306097,
-0.0178113207,
-0.0258908607,
-0.3486144543,
-0.2984479368,
0.2000668794,
-0.3020641208,
-0.2819244266,
0.239293173,
0.1354365349,
0.4544389248,
0.0401276536,
-0.3937390447,
0.5808199048,
-0.1391111463,
-0.114262931,
0.1804575324,
0.3090637326,
-0.0905660689,
0.1654046625,
-0.0495853201,
-0.1657476574,
-0.2646008134,
-0.1806015223,
-0.2320664823,
0.1591045558,
-0.1456698477,
0.0627861172,
0.0104182102,
0.8047190905,
0.0654787272,
-0.2943532467,
0.1827454716,
0.1267127097,
0.2218525857,
-0.00069613,
-0.5895356536,
0.2010114342,
-0.0986169949,
0.2215836346,
0.3626621962,
0.043290507,
0.1737744957,
-0.1139221862,
0.1939599067,
-0.1887793839,
-0.3513716459,
-0.1281633228,
0.2005106509,
0.3849680722,
0.0900529847,
0.1219887733,
0.0273913853,
-0.0954924673,
-0.0095354188,
0.3547011018,
0.1222475618,
0.0885328799,
-0.1050637513,
-0.0560124964,
-0.2369754314,
0.0535889491,
-0.1932055205,
0.4575622976,
-0.0324501917,
-0.1230475605,
-0.0423156507,
-0.0182905514,
0.5026885867,
0.1967384666,
0.3927700222,
0.1022481918,
-0.4248225689,
-0.2393002212,
-0.1275757402,
0.0902223736,
-0.0735772923,
0.1285208017,
0.1846210957,
-0.2020291835,
-0.5072084069,
0.2025790662,
0.1107910275,
-0.1888127476,
-0.1198270246,
-0.1453296542,
0.0249492191,
-0.2902212739,
-0.0777403265,
-0.2248144597,
0.1797916144,
-0.4049387276,
-0.0239726696,
-0.1545185894,
-0.0788379833,
0.1511587799,
0.065721795,
0.0934650525,
0.0443000235,
0.4923815429,
0.3122970462,
0.3200490773,
0.5650812387,
0.6159528494,
-0.1010638475,
0.0344184749,
0.083505556,
0.0163795929,
0.0522826947,
0.2831605077,
-0.0781764761,
0.111100398,
0.0438613966,
0.1107480004,
-0.596083045,
0.1240011081,
0.2019081116,
0.0680200979,
-0.357242316,
-0.0997594744,
0.1946077496,
0.0268736612,
0.2006979585,
0.176009953,
0.1702609509,
-0.3972955346,
-0.4666158855,
0.0168192126,
0.494731307,
-0.0735876039,
0.2222426087,
-0.1423189491,
-0.1103768498,
0.0566483587,
0.2778767943,
-0.1172654331,
-0.191495344,
0.17908521,
0.031930849,
-0.1585559994,
0.0860941932,
0.1152570173,
-0.0740364119,
0.2761103511,
0.0473109894,
0.5473354459,
0.1399032772,
0.0827617273,
-0.133387953,
-0.2570033967,
-0.2534720302,
0.1469008476,
-0.0401019156,
0.3355661333,
-0.2314385772,
-0.1019252166,
0.3119748831,
-0.0665351152,
-0.2452949286,
0.0065799658,
-0.6495984197,
0.1033414751,
-0.0357613713,
-0.10310179,
0.3692241311,
-0.2313643992,
0.1811164171,
0.3199763894,
-0.1902632564,
0.1010997146,
-0.3137946725,
0.0383314714,
-0.2302721739,
0.2646710277,
0.100801602,
-0.0336171463,
-0.0603651293,
-0.1086392254,
-0.0407439806,
-0.0539292656,
0.0338525698,
0.0507711321,
-0.055291675,
-0.0493356995,
-0.3194901943,
0.0118200779,
0.0131510403,
-0.1511107981,
0.1543609947,
0.0290809143,
-0.0549285747,
0.3291853666,
-0.0549550913,
-0.1067547947,
-0.0585502312,
0.4145899415,
-0.2623611391,
0.0229863096,
0.3038801551,
0.4151067436,
-0.0175479371,
-0.2813272178,
-0.068515338,
0.0908065215,
-0.2448112816,
0.2701679766,
0.1164216697,
-0.0899813846,
0.3801681697,
0.5611447096,
0.3422604203,
-0.1498253495,
0.0122463014,
-0.2868365049,
-0.1911179572,
0.0608149767,
-0.1435581595,
0.1625947207,
0.0277144164,
0.4404928386,
-0.1747052372,
0.3926444352,
-0.3059358597,
0.2749380171,
-0.0947573632,
-0.0000581009,
0.2123727202,
-0.1585533321,
0.1591584831,
-0.0921345279,
0.0412249081,
-0.0340269804,
-0.5342017412,
-0.2137607485,
-0.1178594679,
0.0945380926,
0.0980820432,
-0.2711147368,
0.1690741181,
-0.1080358848,
0.0807956308,
-0.1697109938,
0.3470470011,
0.0660691932,
-0.1222604066,
0.0229023024,
0.0703014061,
0.0658249035,
-0.168961376,
0.1390582174,
0.071453236,
-0.067727305,
-0.0049926243,
-0.0410778932,
0.0441779383,
0.0208380353,
0.1150302738,
0.010286944,
-0.0216587577,
0.1182221621,
0.5094001293,
-0.3056087792,
-0.2525113225,
0.2638110518,
0.2856665254,
0.1686182767,
0.0513614826,
0.0614276715,
-0.1690222174,
0.1712697595,
-0.2953844368,
-0.079539448,
-0.037757352,
0.1637817472,
0.0857126117,
0.0499725528,
0.3570750058,
-0.2151892781,
-0.2337027043,
0.3549879789,
0.3251189888,
-0.1116919667,
0.1748535186,
0.4612628818,
0.0847790688,
0.2086673379,
0.1075343117,
-0.1653203368,
0.1047749072,
0.3765079081,
-0.2396266609,
0.6803705692,
0.0646546334,
0.0063732942,
0.2407554835,
-0.4143281877,
0.1107712686,
0.2690978944,
-0.1568296105,
0.0244204495,
-0.2630804479,
0.1377332807,
-0.340200752,
-0.2731530964,
-0.1855366379,
0.1984927952,
-0.0655510426,
-0.1254136115,
-0.2556760013,
-0.1166857928,
-0.0780742839,
0.0273625441,
-0.0752226338,
-0.102267839,
0.2595923841,
0.1732609868,
-0.1564744711,
-0.4046474993,
-0.3694780767,
0.0161440447,
-0.0983203799,
-0.1228211895,
0.2560080886,
0.4048369229,
-0.2123368531,
0.1384949088,
0.4051624238,
0.4715677798,
0.3013881147,
-0.0192861278,
-0.0860338286,
0.0871974751,
-0.0851399228,
-0.277500242,
0.4197262824,
-0.2568071783,
-0.1573419273,
0.0049491497,
0.1699632853,
-0.1862419844,
-0.3644251525,
0.1278569251,
-0.3126043379,
-0.283562094,
0.1925963014,
-0.0886966735,
-0.057648968,
-0.0464048795,
0.3801314831,
-0.046357248,
0.2271073759,
0.6677178741,
-0.0275355075,
0.1280926317,
-0.2423587143,
0.1004885733,
0.0852619261,
0.7199056745,
0.2930561304,
-0.0817144364,
-0.2865350842,
-0.5099944472,
-0.781591475,
0.0482606329,
0.1542912275,
0.4362872243,
0.0736526847,
0.0439880788,
0.1192256212,
-0.0225300677,
0.1537164599,
0.122018531,
0.2925756872,
-0.2151332051,
-0.3548650742,
0.1796958297,
-0.0950154215,
-0.1906717718,
-0.0053812056,
-0.3220203221,
0.1466881186,
-0.2788924873,
0.0247031543,
-0.2417235225,
0.4235900044,
0.0029750341,
0.2875812948,
0.2188120335,
0.0880906209,
0.306371212,
0.0107986862,
-0.0630574971,
-0.4197324216,
-0.3112782538,
0.0145373214,
0.086073719,
-0.1074680313,
0.3646840751,
-0.0537012331,
-0.2685219049,
-0.4432088733,
0.105085738,
0.1143685207,
0.2023359239,
-0.1769941002,
0.2222106755,
-0.2147683799,
-0.1733143181,
0.3443756104,
0.4282422364,
-0.0292371009,
0.187837109,
-0.2106768787,
-0.3873318434,
0.2546338141,
-0.2887780964,
-0.1629845053,
-0.4629841745,
0.1772363037,
-0.0954382494,
-0.1698632091,
-0.4217801392,
0.152843684,
0.1712171882,
-0.1016815007,
-0.2147470266,
0.3726131022,
-0.0460542291,
-0.0684141368,
-0.0185813867,
0.1694672555,
0.1803848594,
-0.3343188167,
-0.1139622703,
-0.0598334782
]
|
https://github.com/huggingface/datasets/issues/1977 | ModuleNotFoundError: No module named 'apache_beam' for wikipedia datasets | I sometimes also get this error with other languages of the same dataset:
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 322, in read_table
stream = stream_from(filename)
File "pyarrow/io.pxi", line 782, in pyarrow.lib.memory_map
File "pyarrow/io.pxi", line 743, in pyarrow.lib.MemoryMappedFile._open
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 99, in pyarrow.lib.check_status
OSError: Memory mapping file failed: Cannot allocate memory
@lhoestq
| Hi
I am trying to run run_mlm.py code [1] of huggingface with following "wikipedia"/ "20200501.aa" dataset:
`python run_mlm.py --model_name_or_path bert-base-multilingual-cased --dataset_name wikipedia --dataset_config_name 20200501.aa --do_train --do_eval --output_dir /tmp/test-mlm --max_seq_length 256
`
I am getting this error, but as per documentation, huggingface dataset provide processed version of this dataset and users can load it without requiring setup extra settings for apache-beam. could you help me please to load this dataset?
Do you think I can run run_ml.py with this dataset? or anyway I could subsample and train the model? I greatly appreciate providing the processed version of all languages for this dataset, which allow the user to use them without setting up apache-beam,. thanks
I really appreciate your help.
@lhoestq
thanks.
[1] https://github.com/huggingface/transformers/blob/master/examples/language-modeling/run_mlm.py
error I get:
```
>>> import datasets
>>> datasets.load_dataset("wikipedia", "20200501.aa")
Downloading and preparing dataset wikipedia/20200501.aa (download: Unknown size, generated: Unknown size, post-processed: Unknown size, total: Unknown size) to /dara/temp/cache_home_2/datasets/wikipedia/20200501.aa/1.0.0/4021357e28509391eab2f8300d9b689e7e8f3a877ebb3d354b01577d497ebc63...
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/dara/temp/libs/anaconda3/envs/codes/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/load.py", line 746, in load_dataset
use_auth_token=use_auth_token,
File "/dara/temp/libs/anaconda3/envs/codes/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 573, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/dara/temp/libs/anaconda3/envs/codes/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 1099, in _download_and_prepare
import apache_beam as beam
ModuleNotFoundError: No module named 'apache_beam'
``` | 55 | ModuleNotFoundError: No module named 'apache_beam' for wikipedia datasets
Hi
I am trying to run run_mlm.py code [1] of huggingface with following "wikipedia"/ "20200501.aa" dataset:
`python run_mlm.py --model_name_or_path bert-base-multilingual-cased --dataset_name wikipedia --dataset_config_name 20200501.aa --do_train --do_eval --output_dir /tmp/test-mlm --max_seq_length 256
`
I am getting this error, but as per documentation, huggingface dataset provide processed version of this dataset and users can load it without requiring setup extra settings for apache-beam. could you help me please to load this dataset?
Do you think I can run run_ml.py with this dataset? or anyway I could subsample and train the model? I greatly appreciate providing the processed version of all languages for this dataset, which allow the user to use them without setting up apache-beam,. thanks
I really appreciate your help.
@lhoestq
thanks.
[1] https://github.com/huggingface/transformers/blob/master/examples/language-modeling/run_mlm.py
error I get:
```
>>> import datasets
>>> datasets.load_dataset("wikipedia", "20200501.aa")
Downloading and preparing dataset wikipedia/20200501.aa (download: Unknown size, generated: Unknown size, post-processed: Unknown size, total: Unknown size) to /dara/temp/cache_home_2/datasets/wikipedia/20200501.aa/1.0.0/4021357e28509391eab2f8300d9b689e7e8f3a877ebb3d354b01577d497ebc63...
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/dara/temp/libs/anaconda3/envs/codes/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/load.py", line 746, in load_dataset
use_auth_token=use_auth_token,
File "/dara/temp/libs/anaconda3/envs/codes/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 573, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/dara/temp/libs/anaconda3/envs/codes/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 1099, in _download_and_prepare
import apache_beam as beam
ModuleNotFoundError: No module named 'apache_beam'
```
I sometimes also get this error with other languages of the same dataset:
File "/dara/libs/anaconda3/envs/code/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/arrow_reader.py", line 322, in read_table
stream = stream_from(filename)
File "pyarrow/io.pxi", line 782, in pyarrow.lib.memory_map
File "pyarrow/io.pxi", line 743, in pyarrow.lib.MemoryMappedFile._open
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 99, in pyarrow.lib.check_status
OSError: Memory mapping file failed: Cannot allocate memory
@lhoestq
| [
-0.2131416649,
-0.3383004069,
0.0015386621,
0.3398338556,
0.2197448462,
0.2354417443,
0.2507149279,
0.2680619955,
0.1814851612,
-0.0144519843,
-0.0359321311,
0.0364904329,
-0.1710566133,
0.1019866467,
0.1627784073,
-0.4244245589,
0.1850164682,
-0.1324692667,
-0.2871963382,
-0.0623329878,
-0.2229190767,
0.3467075825,
-0.2787268162,
0.0804545656,
-0.0931129828,
-0.111704357,
-0.0233220216,
0.1777574867,
-0.0540726557,
-0.1476042718,
0.1096978858,
-0.2670558989,
0.2810364962,
0.3396107852,
-0.0001204881,
0.0703729987,
0.3882136047,
-0.2105151266,
-0.3313601017,
-0.3505054414,
0.1729955226,
-0.1294175684,
0.1381458491,
-0.2263076454,
-0.2553459704,
-0.1438835412,
0.1525820047,
-0.2568328977,
0.6282419562,
-0.0624547228,
0.1526159644,
0.3100944757,
0.3425410092,
-0.0296260882,
0.1350964904,
0.2360667288,
-0.0238422342,
0.3365867138,
-0.1699713916,
-0.1384140402,
0.1368695498,
0.5407435298,
-0.2211785167,
-0.0125281541,
0.4623476565,
-0.2002918273,
0.0987882093,
-0.5092286468,
0.1167537495,
0.2758408189,
0.5031312108,
-0.2679271102,
-0.1555138081,
-0.0499551482,
0.0338076577,
-0.0353640504,
0.023285253,
0.2298908532,
-0.1179501489,
0.0734568313,
0.229557395,
-0.3673404157,
-0.1967660785,
0.2305391878,
0.2532693744,
0.2152471244,
-0.0669513047,
0.2225007564,
0.1748568267,
-0.1025936827,
-0.4621139467,
-0.0402749553,
0.1561260819,
0.4422610998,
-0.2312315702,
0.0028232932,
-0.1472998857,
0.2895690501,
0.2478105128,
-0.1703074872,
-0.4538455904,
0.1153054535,
0.2710077167,
0.0321764164,
0.0142413592,
0.0148337753,
0.1804623604,
-0.1404561698,
0.2022215873,
0.1521081179,
0.0284995586,
0.0471680984,
-0.0834325403,
-0.0926122218,
-0.6663611531,
-0.0144305546,
0.257178098,
-0.1104111746,
-0.1701252162,
0.0090118395,
-0.5486821532,
-0.2558386028,
-0.1178340986,
0.491484195,
-0.0876235962,
-0.0432361588,
0.3066844344,
0.2725152969,
-0.319604367,
-0.332947135,
-0.0259875488,
0.3970682025,
-0.404242605,
0.2580560148,
0.1624426395,
-0.1852098256,
0.3300161958,
-0.0969915166,
0.0612081364,
-0.0126666296,
0.0938327089,
0.0160335619,
-0.2645788193,
0.1376617104,
0.2601521015,
0.2935318649,
0.3347854912,
-0.1072972193,
-0.1303371787,
-0.0130058099,
-0.2017823905,
-0.0785867125,
0.0849884227,
0.0104480088,
-0.1748300046,
0.151697129,
-0.1632313281,
0.2782360613,
-0.0621966086,
0.0589514636,
-0.0485334359,
0.2518559992,
-0.0731161907,
-0.1545421332,
0.4467759728,
0.7152792215,
-0.0924545079,
-0.1832816005,
-0.1248654649,
0.0888796672,
-0.1330817491,
0.0378331505,
-0.0201485157,
0.2038293928,
-0.1944644004,
0.0074123894,
0.2688696682,
-0.4080124795,
0.0281282924,
0.0515701473,
0.0758029073,
0.0689503551,
0.1730378866,
-0.1063593179,
-0.2876467705,
-0.0430138856,
-0.0504935049,
0.2006358802,
0.1387093812,
-0.0972391069,
-0.1221535876,
-0.260840863,
0.0938871577,
0.2339618057,
0.1046054363,
-0.0214353539,
0.0598470643,
0.5199070573,
0.2253657281,
-0.1468619555,
0.1068721116,
0.3651062846,
-0.1032072827,
0.2124210894,
0.0801008865,
-0.2059350163,
-0.1896689534,
0.0508288778,
-0.2155384123,
0.5097806454,
-0.1102501899,
-0.0496580675,
-0.206275776,
-0.0623014458,
-0.1766988933,
-0.5256923437,
0.0174632501,
-0.1082241461,
0.1392479092,
0.3834109604,
-0.1024010554,
0.2250567228,
0.0055662254,
0.199066624,
-0.853918016,
0.1584816426,
-0.1625043899,
0.0418301448,
0.118834652,
0.2998980284,
0.14185597,
-0.2450332195,
0.1450187415,
0.2261150628,
0.0557803772,
0.012418123,
0.0420553535,
-0.1097353026,
0.1478801966,
-0.3845857978,
0.2552293837,
0.0223891865,
0.0874387845,
0.0594491214,
0.0067110807,
0.2668875158,
0.1542762071,
0.3922146559,
0.1603033245,
0.1677393168,
-0.0652834848,
0.0154549675,
-0.2106283456,
-0.3001225889,
0.3233879209,
0.1925315708,
0.331528306,
-0.2222322822,
-0.119722791,
-0.3948611021,
0.2577238083,
-0.0251156911,
0.2270759344,
-0.0560161024,
-0.6535448432,
0.290453434,
0.1373187602,
-0.2333567888,
0.1929881126,
0.2338313609,
-0.17620112,
0.2047182471,
0.1758314371,
0.0648408309,
0.2654977441,
0.0882546082,
0.4785860777,
-0.0819544345,
-0.229449138,
-0.1114422828,
-0.2178026736,
-0.1793962419,
-0.2691537738,
0.1448301375,
-0.3526335061,
0.1320127398,
-0.2323406637,
-0.5582596064,
-0.3051200509,
-0.0217384007,
-0.5030117035,
-0.0974111781,
-0.3454746008,
0.153003782,
0.1994352788,
0.4281542301,
0.2881692052,
-0.0364837497,
0.0833427757,
-0.1567297429,
-0.18805103,
-0.1502946466,
-0.2787017524,
-0.0067598182,
0.375251472,
0.13934277,
0.1550536454,
-0.2120733708,
-0.0861390531,
-0.0383539759,
-0.4270922542,
0.2244887203,
-0.2542715669,
0.2628421485,
0.1885616034,
0.4130572081,
-0.1757038385,
-0.0502506495,
0.3604338467,
0.0225338805,
-0.0979226083,
-0.0710495338,
0.0758106336,
0.0790890753,
0.0070316931,
-0.2876693606,
-0.2747098207,
-0.3001061678,
0.1849594861,
0.1132887825,
-0.0709093213,
0.3787706196,
0.2008130103,
0.1534817964,
-0.0516653694,
0.0577603392,
-0.1449173242,
0.0399071202,
0.3708280325,
-0.2393714935,
-0.3559532166,
0.186473906,
-0.1147245094,
0.2194797099,
-0.0391897149,
-0.2681618333,
-0.1775779873,
0.335468024,
-0.1826423556,
-0.0108241802,
0.3228735328,
0.2339254767,
-0.134177193,
0.1562602222,
-0.1174856052,
-0.0410773009,
-0.0801703632,
-0.4929059148,
0.3673176765,
0.2488036752,
0.6314402223,
-0.0803146362,
0.8895263672,
0.2763773799,
0.2054400146,
0.1781022102,
-0.0261579826,
0.3253340423,
-0.0767208859,
-0.2912581861,
-0.1696088314,
0.0166211929,
0.1385062039,
0.3068870902,
-0.0287207719,
0.0690139532,
-0.5301668048,
-0.2567857206,
-0.1413566321,
-0.2349941283,
0.0289410818,
-0.1157509238,
0.4020375013,
0.0677075386,
0.2030599862,
0.063127704,
-0.0334786661,
0.3315420449,
0.5468326211,
0.0733549744,
0.1779472977,
-0.0101675307,
-0.19918257,
-0.5310757756,
0.402153492,
-0.1498969942,
0.0007156951,
-0.0902605578,
-0.1064204499,
0.1710890383,
0.0208135545,
0.7178603411,
-0.0888773575,
-0.0936146602,
0.1358969659,
0.0015434416,
-0.7305617332,
0.1050548106,
0.0151007613,
0.1899867207,
0.1572464406,
0.2613230646,
-0.4709852338,
-0.1298937798,
0.4364516437,
0.1856457442,
-0.1291806698,
-0.1521936953,
-0.524649322,
-0.1736597717,
-0.4372418225,
-0.1901435107,
-0.0122952703,
0.1366354823,
0.1450325847,
0.3733562231,
0.0267636552,
-0.1719459444,
0.261996448,
0.2708523273,
0.3428228796,
-0.0041509569,
-0.1550677866,
0.1976630092,
0.3420522809,
-0.0263307691,
0.1929225028,
-0.0081906673,
-0.4109749794,
-0.0481522568,
0.0178303923,
0.232472524,
0.1311828792,
-0.1344868541,
0.1006972343,
0.193182379,
-0.0921951383,
-0.1482952982,
-0.095378153,
0.2387634218,
0.2665477097,
-0.3958498538,
-0.4166851938,
0.6590349078,
0.0648799613,
0.056840606,
0.1441072077,
0.2705354691,
-0.329798907,
0.3083681464,
0.1000933126,
1.0329893827,
-0.0303209275,
0.1867604256,
0.2142025232,
0.161411494,
0.8284100294,
-0.4468649626,
0.1807177961,
-0.3208567798,
0.1913253218,
-0.0089976639,
-0.0728423446,
0.4384716451,
0.0767246857,
-0.1231358796,
0.3307365179,
0.220532611,
0.0837882087,
-0.1420511305,
0.4586179852,
-0.0194508415,
-0.221965313,
-0.4577874839,
0.0059212167,
-0.1063209251,
0.6052086949,
-0.2764022946,
-0.1886196584,
-0.2304378152,
-0.2535760999,
-0.1999851167,
-0.0153511558,
-0.353202492,
0.2437591851,
-0.1092239395,
-0.2311705351,
0.3377099037,
0.2006302029,
0.2454079092,
0.0233234372,
-0.333147645,
0.1841921955,
-0.3711703122,
-0.4309198558,
-0.200715676,
0.0908434391,
0.321277082,
-0.1738762856,
-0.1705291271,
0.0283663031,
-0.0744693652,
0.0023994118,
-0.1516768187,
-0.1976521462,
0.2312407047,
0.041747205,
-0.1732558012,
0.2482137233,
0.1364868283,
0.1078021303,
0.0466101021,
-0.1768838316,
-0.1747900993,
-0.059520252,
0.18114838,
-0.1508109421,
-0.0135540226,
0.4774491191,
0.151592344,
-0.0545032732,
0.5850342512,
0.4320332706,
-0.1466630101,
-0.1480634958,
0.115029484,
0.0594774969,
-0.3519894481,
-0.0794998035,
0.3436746597,
0.2288253605,
-0.1090680659,
0.0155703919,
0.0660212934,
-0.2205021381,
0.1175460741,
-0.4755689502,
-0.1650405526,
0.4451123178,
-0.2226314992,
-0.1648505479,
0.219207108,
0.2640363276,
0.222073108,
-0.0910978094,
-0.1593146026,
-0.1072035059,
0.1454123408,
0.0105217081,
0.5708833933,
0.0572470501,
0.1781498045,
0.1490950882,
0.044193022,
0.0211146772,
-0.2832328975,
-0.0636304393,
-0.0720100701,
0.2172734141,
-0.1876896471,
-0.1258061975,
0.0418042541,
-0.2209443301,
-0.0505201109,
-0.186130181,
0.0226196628,
-0.052978605,
0.0284199174,
0.0480480604,
0.0441343524,
0.2348094434,
-0.4368966222,
0.0437429398,
-0.0688095465,
0.1023069099,
0.2306391597,
-0.0978876576,
0.3817754686,
0.0426770635,
-0.3169854283,
0.0096645635,
0.0188738815,
-0.0928129479,
0.2114265412,
0.0173063576,
-0.1756637394,
-0.0178819075,
0.0450213552,
0.2304659933,
-0.1178768501,
0.0271784104,
0.2184097767,
0.0492902808,
-0.4587969184,
0.3201699257,
0.4787882566,
-0.2735526264,
-0.0876099914,
0.2480544001,
0.0477452911,
0.3263322711,
0.0658335462,
0.1816514581,
0.2887360752,
0.1045155674,
0.3736536801,
0.0241867714,
0.123909153,
0.1329518259,
0.2444556504,
0.1555862129,
0.2112367302,
0.4075266123,
-0.2197608352,
0.2934307754,
-0.2903066874,
0.0693987682,
-0.0584797524,
-0.1634107679,
0.1687479019,
0.3123416603,
0.0832755938,
0.1723278165,
-0.2653712332,
0.4888257086,
-0.0398290679,
-0.2026305944,
-0.1932529211,
0.2618727386,
-0.0716300234,
-0.1267793328,
-0.1107864156,
-0.221742332,
-0.1446861923,
-0.0342083871,
0.1442166716,
-0.6080905199,
0.1658253819,
0.1780466884,
-0.132517755,
-0.1697183549,
-0.1060798243,
0.2342265844,
0.1096986011,
-0.0739863664,
-0.0205644798,
0.0220542289,
0.0508319512,
-0.0317689814,
0.0707947835,
0.2845673859,
0.069499515,
-0.3261436224,
-0.0886076391,
0.0281690601,
0.0773353204,
0.154069379,
-0.0325724706,
0.1480217576,
0.2344674468,
0.1302424818,
0.0422345214,
-0.0877720937,
-0.1766923964,
0.1153909341,
0.0173509438,
-0.3497007787,
-0.1444837153,
-0.5136171579,
0.1191736534,
-0.3950395584,
0.1520347148,
-0.6309113503,
0.3177129924,
0.2420934588,
0.1043874994,
-0.2357273996,
-0.2944582403,
-0.0183673538,
-0.2088821679,
0.4825663567,
0.2201554924,
0.1175154746,
-0.2192919254,
-0.349817872,
-0.5625337958,
-0.0253222752,
-0.1389906704,
-0.0824832618,
-0.2705151141,
-0.004103241,
0.2746977508,
0.2762577236,
-0.0568135418,
-0.0429307111,
-0.0837088376,
0.0761818588,
-0.1995842159,
-0.2112318575,
-0.3830579519,
0.1804931462,
-0.1026849002,
-0.0495566353,
-0.0805135667,
-0.0298240446,
-0.1119440049,
-0.2530979216,
-0.1765733808,
0.1710342169,
-0.1491255015,
0.3884041011,
-0.0165547654,
0.3359569013,
0.0793647543,
-0.1874969751,
-0.1123814583,
0.0434571691,
-0.0540188327,
0.3798465133,
0.063410148,
0.2255601734,
-0.2784628272,
-0.3539013863,
-0.3619383574,
0.5960182548,
0.1159186289,
-0.0575565882,
-0.3417953849,
0.1050299555,
-0.0232627857,
0.1765860617,
-0.0566633046,
0.2489113957,
-0.1592449248,
0.0920144394,
-0.4346443415,
-0.0472102985,
0.5104726553,
-0.8678762317,
-0.2605216503,
0.003188004,
0.0732160285,
-0.0388209298,
-0.2040878981,
-0.6355014443,
0.1892228127,
0.3016796112,
0.1750744879,
-0.0031793572,
0.227770552,
-0.1221882775,
-0.0525792949,
-0.0560737103,
-0.3247557878,
-0.0901469663,
-0.1945209801,
0.0034506992,
-0.2350439876
]
|
https://github.com/huggingface/datasets/issues/1977 | ModuleNotFoundError: No module named 'apache_beam' for wikipedia datasets | Hi ! Thanks for reporting
Some wikipedia configurations do require the user to have `apache_beam` in order to parse the wikimedia data.
On the other hand regarding your second issue
```
OSError: Memory mapping file failed: Cannot allocate memory
```
I've never experienced this, can you open a new issue for this specific error and provide more details please ?
For example what script did you use to get this, what language did you use, what's your environment details (os, python version, pyarrow version).. | Hi
I am trying to run run_mlm.py code [1] of huggingface with following "wikipedia"/ "20200501.aa" dataset:
`python run_mlm.py --model_name_or_path bert-base-multilingual-cased --dataset_name wikipedia --dataset_config_name 20200501.aa --do_train --do_eval --output_dir /tmp/test-mlm --max_seq_length 256
`
I am getting this error, but as per documentation, huggingface dataset provide processed version of this dataset and users can load it without requiring setup extra settings for apache-beam. could you help me please to load this dataset?
Do you think I can run run_ml.py with this dataset? or anyway I could subsample and train the model? I greatly appreciate providing the processed version of all languages for this dataset, which allow the user to use them without setting up apache-beam,. thanks
I really appreciate your help.
@lhoestq
thanks.
[1] https://github.com/huggingface/transformers/blob/master/examples/language-modeling/run_mlm.py
error I get:
```
>>> import datasets
>>> datasets.load_dataset("wikipedia", "20200501.aa")
Downloading and preparing dataset wikipedia/20200501.aa (download: Unknown size, generated: Unknown size, post-processed: Unknown size, total: Unknown size) to /dara/temp/cache_home_2/datasets/wikipedia/20200501.aa/1.0.0/4021357e28509391eab2f8300d9b689e7e8f3a877ebb3d354b01577d497ebc63...
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/dara/temp/libs/anaconda3/envs/codes/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/load.py", line 746, in load_dataset
use_auth_token=use_auth_token,
File "/dara/temp/libs/anaconda3/envs/codes/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 573, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/dara/temp/libs/anaconda3/envs/codes/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 1099, in _download_and_prepare
import apache_beam as beam
ModuleNotFoundError: No module named 'apache_beam'
``` | 84 | ModuleNotFoundError: No module named 'apache_beam' for wikipedia datasets
Hi
I am trying to run run_mlm.py code [1] of huggingface with following "wikipedia"/ "20200501.aa" dataset:
`python run_mlm.py --model_name_or_path bert-base-multilingual-cased --dataset_name wikipedia --dataset_config_name 20200501.aa --do_train --do_eval --output_dir /tmp/test-mlm --max_seq_length 256
`
I am getting this error, but as per documentation, huggingface dataset provide processed version of this dataset and users can load it without requiring setup extra settings for apache-beam. could you help me please to load this dataset?
Do you think I can run run_ml.py with this dataset? or anyway I could subsample and train the model? I greatly appreciate providing the processed version of all languages for this dataset, which allow the user to use them without setting up apache-beam,. thanks
I really appreciate your help.
@lhoestq
thanks.
[1] https://github.com/huggingface/transformers/blob/master/examples/language-modeling/run_mlm.py
error I get:
```
>>> import datasets
>>> datasets.load_dataset("wikipedia", "20200501.aa")
Downloading and preparing dataset wikipedia/20200501.aa (download: Unknown size, generated: Unknown size, post-processed: Unknown size, total: Unknown size) to /dara/temp/cache_home_2/datasets/wikipedia/20200501.aa/1.0.0/4021357e28509391eab2f8300d9b689e7e8f3a877ebb3d354b01577d497ebc63...
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/dara/temp/libs/anaconda3/envs/codes/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/load.py", line 746, in load_dataset
use_auth_token=use_auth_token,
File "/dara/temp/libs/anaconda3/envs/codes/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 573, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/dara/temp/libs/anaconda3/envs/codes/lib/python3.7/site-packages/datasets-1.3.0-py3.7.egg/datasets/builder.py", line 1099, in _download_and_prepare
import apache_beam as beam
ModuleNotFoundError: No module named 'apache_beam'
```
Hi ! Thanks for reporting
Some wikipedia configurations do require the user to have `apache_beam` in order to parse the wikimedia data.
On the other hand regarding your second issue
```
OSError: Memory mapping file failed: Cannot allocate memory
```
I've never experienced this, can you open a new issue for this specific error and provide more details please ?
For example what script did you use to get this, what language did you use, what's your environment details (os, python version, pyarrow version).. | [
-0.2131416649,
-0.3383004069,
0.0015386621,
0.3398338556,
0.2197448462,
0.2354417443,
0.2507149279,
0.2680619955,
0.1814851612,
-0.0144519843,
-0.0359321311,
0.0364904329,
-0.1710566133,
0.1019866467,
0.1627784073,
-0.4244245589,
0.1850164682,
-0.1324692667,
-0.2871963382,
-0.0623329878,
-0.2229190767,
0.3467075825,
-0.2787268162,
0.0804545656,
-0.0931129828,
-0.111704357,
-0.0233220216,
0.1777574867,
-0.0540726557,
-0.1476042718,
0.1096978858,
-0.2670558989,
0.2810364962,
0.3396107852,
-0.0001204881,
0.0703729987,
0.3882136047,
-0.2105151266,
-0.3313601017,
-0.3505054414,
0.1729955226,
-0.1294175684,
0.1381458491,
-0.2263076454,
-0.2553459704,
-0.1438835412,
0.1525820047,
-0.2568328977,
0.6282419562,
-0.0624547228,
0.1526159644,
0.3100944757,
0.3425410092,
-0.0296260882,
0.1350964904,
0.2360667288,
-0.0238422342,
0.3365867138,
-0.1699713916,
-0.1384140402,
0.1368695498,
0.5407435298,
-0.2211785167,
-0.0125281541,
0.4623476565,
-0.2002918273,
0.0987882093,
-0.5092286468,
0.1167537495,
0.2758408189,
0.5031312108,
-0.2679271102,
-0.1555138081,
-0.0499551482,
0.0338076577,
-0.0353640504,
0.023285253,
0.2298908532,
-0.1179501489,
0.0734568313,
0.229557395,
-0.3673404157,
-0.1967660785,
0.2305391878,
0.2532693744,
0.2152471244,
-0.0669513047,
0.2225007564,
0.1748568267,
-0.1025936827,
-0.4621139467,
-0.0402749553,
0.1561260819,
0.4422610998,
-0.2312315702,
0.0028232932,
-0.1472998857,
0.2895690501,
0.2478105128,
-0.1703074872,
-0.4538455904,
0.1153054535,
0.2710077167,
0.0321764164,
0.0142413592,
0.0148337753,
0.1804623604,
-0.1404561698,
0.2022215873,
0.1521081179,
0.0284995586,
0.0471680984,
-0.0834325403,
-0.0926122218,
-0.6663611531,
-0.0144305546,
0.257178098,
-0.1104111746,
-0.1701252162,
0.0090118395,
-0.5486821532,
-0.2558386028,
-0.1178340986,
0.491484195,
-0.0876235962,
-0.0432361588,
0.3066844344,
0.2725152969,
-0.319604367,
-0.332947135,
-0.0259875488,
0.3970682025,
-0.404242605,
0.2580560148,
0.1624426395,
-0.1852098256,
0.3300161958,
-0.0969915166,
0.0612081364,
-0.0126666296,
0.0938327089,
0.0160335619,
-0.2645788193,
0.1376617104,
0.2601521015,
0.2935318649,
0.3347854912,
-0.1072972193,
-0.1303371787,
-0.0130058099,
-0.2017823905,
-0.0785867125,
0.0849884227,
0.0104480088,
-0.1748300046,
0.151697129,
-0.1632313281,
0.2782360613,
-0.0621966086,
0.0589514636,
-0.0485334359,
0.2518559992,
-0.0731161907,
-0.1545421332,
0.4467759728,
0.7152792215,
-0.0924545079,
-0.1832816005,
-0.1248654649,
0.0888796672,
-0.1330817491,
0.0378331505,
-0.0201485157,
0.2038293928,
-0.1944644004,
0.0074123894,
0.2688696682,
-0.4080124795,
0.0281282924,
0.0515701473,
0.0758029073,
0.0689503551,
0.1730378866,
-0.1063593179,
-0.2876467705,
-0.0430138856,
-0.0504935049,
0.2006358802,
0.1387093812,
-0.0972391069,
-0.1221535876,
-0.260840863,
0.0938871577,
0.2339618057,
0.1046054363,
-0.0214353539,
0.0598470643,
0.5199070573,
0.2253657281,
-0.1468619555,
0.1068721116,
0.3651062846,
-0.1032072827,
0.2124210894,
0.0801008865,
-0.2059350163,
-0.1896689534,
0.0508288778,
-0.2155384123,
0.5097806454,
-0.1102501899,
-0.0496580675,
-0.206275776,
-0.0623014458,
-0.1766988933,
-0.5256923437,
0.0174632501,
-0.1082241461,
0.1392479092,
0.3834109604,
-0.1024010554,
0.2250567228,
0.0055662254,
0.199066624,
-0.853918016,
0.1584816426,
-0.1625043899,
0.0418301448,
0.118834652,
0.2998980284,
0.14185597,
-0.2450332195,
0.1450187415,
0.2261150628,
0.0557803772,
0.012418123,
0.0420553535,
-0.1097353026,
0.1478801966,
-0.3845857978,
0.2552293837,
0.0223891865,
0.0874387845,
0.0594491214,
0.0067110807,
0.2668875158,
0.1542762071,
0.3922146559,
0.1603033245,
0.1677393168,
-0.0652834848,
0.0154549675,
-0.2106283456,
-0.3001225889,
0.3233879209,
0.1925315708,
0.331528306,
-0.2222322822,
-0.119722791,
-0.3948611021,
0.2577238083,
-0.0251156911,
0.2270759344,
-0.0560161024,
-0.6535448432,
0.290453434,
0.1373187602,
-0.2333567888,
0.1929881126,
0.2338313609,
-0.17620112,
0.2047182471,
0.1758314371,
0.0648408309,
0.2654977441,
0.0882546082,
0.4785860777,
-0.0819544345,
-0.229449138,
-0.1114422828,
-0.2178026736,
-0.1793962419,
-0.2691537738,
0.1448301375,
-0.3526335061,
0.1320127398,
-0.2323406637,
-0.5582596064,
-0.3051200509,
-0.0217384007,
-0.5030117035,
-0.0974111781,
-0.3454746008,
0.153003782,
0.1994352788,
0.4281542301,
0.2881692052,
-0.0364837497,
0.0833427757,
-0.1567297429,
-0.18805103,
-0.1502946466,
-0.2787017524,
-0.0067598182,
0.375251472,
0.13934277,
0.1550536454,
-0.2120733708,
-0.0861390531,
-0.0383539759,
-0.4270922542,
0.2244887203,
-0.2542715669,
0.2628421485,
0.1885616034,
0.4130572081,
-0.1757038385,
-0.0502506495,
0.3604338467,
0.0225338805,
-0.0979226083,
-0.0710495338,
0.0758106336,
0.0790890753,
0.0070316931,
-0.2876693606,
-0.2747098207,
-0.3001061678,
0.1849594861,
0.1132887825,
-0.0709093213,
0.3787706196,
0.2008130103,
0.1534817964,
-0.0516653694,
0.0577603392,
-0.1449173242,
0.0399071202,
0.3708280325,
-0.2393714935,
-0.3559532166,
0.186473906,
-0.1147245094,
0.2194797099,
-0.0391897149,
-0.2681618333,
-0.1775779873,
0.335468024,
-0.1826423556,
-0.0108241802,
0.3228735328,
0.2339254767,
-0.134177193,
0.1562602222,
-0.1174856052,
-0.0410773009,
-0.0801703632,
-0.4929059148,
0.3673176765,
0.2488036752,
0.6314402223,
-0.0803146362,
0.8895263672,
0.2763773799,
0.2054400146,
0.1781022102,
-0.0261579826,
0.3253340423,
-0.0767208859,
-0.2912581861,
-0.1696088314,
0.0166211929,
0.1385062039,
0.3068870902,
-0.0287207719,
0.0690139532,
-0.5301668048,
-0.2567857206,
-0.1413566321,
-0.2349941283,
0.0289410818,
-0.1157509238,
0.4020375013,
0.0677075386,
0.2030599862,
0.063127704,
-0.0334786661,
0.3315420449,
0.5468326211,
0.0733549744,
0.1779472977,
-0.0101675307,
-0.19918257,
-0.5310757756,
0.402153492,
-0.1498969942,
0.0007156951,
-0.0902605578,
-0.1064204499,
0.1710890383,
0.0208135545,
0.7178603411,
-0.0888773575,
-0.0936146602,
0.1358969659,
0.0015434416,
-0.7305617332,
0.1050548106,
0.0151007613,
0.1899867207,
0.1572464406,
0.2613230646,
-0.4709852338,
-0.1298937798,
0.4364516437,
0.1856457442,
-0.1291806698,
-0.1521936953,
-0.524649322,
-0.1736597717,
-0.4372418225,
-0.1901435107,
-0.0122952703,
0.1366354823,
0.1450325847,
0.3733562231,
0.0267636552,
-0.1719459444,
0.261996448,
0.2708523273,
0.3428228796,
-0.0041509569,
-0.1550677866,
0.1976630092,
0.3420522809,
-0.0263307691,
0.1929225028,
-0.0081906673,
-0.4109749794,
-0.0481522568,
0.0178303923,
0.232472524,
0.1311828792,
-0.1344868541,
0.1006972343,
0.193182379,
-0.0921951383,
-0.1482952982,
-0.095378153,
0.2387634218,
0.2665477097,
-0.3958498538,
-0.4166851938,
0.6590349078,
0.0648799613,
0.056840606,
0.1441072077,
0.2705354691,
-0.329798907,
0.3083681464,
0.1000933126,
1.0329893827,
-0.0303209275,
0.1867604256,
0.2142025232,
0.161411494,
0.8284100294,
-0.4468649626,
0.1807177961,
-0.3208567798,
0.1913253218,
-0.0089976639,
-0.0728423446,
0.4384716451,
0.0767246857,
-0.1231358796,
0.3307365179,
0.220532611,
0.0837882087,
-0.1420511305,
0.4586179852,
-0.0194508415,
-0.221965313,
-0.4577874839,
0.0059212167,
-0.1063209251,
0.6052086949,
-0.2764022946,
-0.1886196584,
-0.2304378152,
-0.2535760999,
-0.1999851167,
-0.0153511558,
-0.353202492,
0.2437591851,
-0.1092239395,
-0.2311705351,
0.3377099037,
0.2006302029,
0.2454079092,
0.0233234372,
-0.333147645,
0.1841921955,
-0.3711703122,
-0.4309198558,
-0.200715676,
0.0908434391,
0.321277082,
-0.1738762856,
-0.1705291271,
0.0283663031,
-0.0744693652,
0.0023994118,
-0.1516768187,
-0.1976521462,
0.2312407047,
0.041747205,
-0.1732558012,
0.2482137233,
0.1364868283,
0.1078021303,
0.0466101021,
-0.1768838316,
-0.1747900993,
-0.059520252,
0.18114838,
-0.1508109421,
-0.0135540226,
0.4774491191,
0.151592344,
-0.0545032732,
0.5850342512,
0.4320332706,
-0.1466630101,
-0.1480634958,
0.115029484,
0.0594774969,
-0.3519894481,
-0.0794998035,
0.3436746597,
0.2288253605,
-0.1090680659,
0.0155703919,
0.0660212934,
-0.2205021381,
0.1175460741,
-0.4755689502,
-0.1650405526,
0.4451123178,
-0.2226314992,
-0.1648505479,
0.219207108,
0.2640363276,
0.222073108,
-0.0910978094,
-0.1593146026,
-0.1072035059,
0.1454123408,
0.0105217081,
0.5708833933,
0.0572470501,
0.1781498045,
0.1490950882,
0.044193022,
0.0211146772,
-0.2832328975,
-0.0636304393,
-0.0720100701,
0.2172734141,
-0.1876896471,
-0.1258061975,
0.0418042541,
-0.2209443301,
-0.0505201109,
-0.186130181,
0.0226196628,
-0.052978605,
0.0284199174,
0.0480480604,
0.0441343524,
0.2348094434,
-0.4368966222,
0.0437429398,
-0.0688095465,
0.1023069099,
0.2306391597,
-0.0978876576,
0.3817754686,
0.0426770635,
-0.3169854283,
0.0096645635,
0.0188738815,
-0.0928129479,
0.2114265412,
0.0173063576,
-0.1756637394,
-0.0178819075,
0.0450213552,
0.2304659933,
-0.1178768501,
0.0271784104,
0.2184097767,
0.0492902808,
-0.4587969184,
0.3201699257,
0.4787882566,
-0.2735526264,
-0.0876099914,
0.2480544001,
0.0477452911,
0.3263322711,
0.0658335462,
0.1816514581,
0.2887360752,
0.1045155674,
0.3736536801,
0.0241867714,
0.123909153,
0.1329518259,
0.2444556504,
0.1555862129,
0.2112367302,
0.4075266123,
-0.2197608352,
0.2934307754,
-0.2903066874,
0.0693987682,
-0.0584797524,
-0.1634107679,
0.1687479019,
0.3123416603,
0.0832755938,
0.1723278165,
-0.2653712332,
0.4888257086,
-0.0398290679,
-0.2026305944,
-0.1932529211,
0.2618727386,
-0.0716300234,
-0.1267793328,
-0.1107864156,
-0.221742332,
-0.1446861923,
-0.0342083871,
0.1442166716,
-0.6080905199,
0.1658253819,
0.1780466884,
-0.132517755,
-0.1697183549,
-0.1060798243,
0.2342265844,
0.1096986011,
-0.0739863664,
-0.0205644798,
0.0220542289,
0.0508319512,
-0.0317689814,
0.0707947835,
0.2845673859,
0.069499515,
-0.3261436224,
-0.0886076391,
0.0281690601,
0.0773353204,
0.154069379,
-0.0325724706,
0.1480217576,
0.2344674468,
0.1302424818,
0.0422345214,
-0.0877720937,
-0.1766923964,
0.1153909341,
0.0173509438,
-0.3497007787,
-0.1444837153,
-0.5136171579,
0.1191736534,
-0.3950395584,
0.1520347148,
-0.6309113503,
0.3177129924,
0.2420934588,
0.1043874994,
-0.2357273996,
-0.2944582403,
-0.0183673538,
-0.2088821679,
0.4825663567,
0.2201554924,
0.1175154746,
-0.2192919254,
-0.349817872,
-0.5625337958,
-0.0253222752,
-0.1389906704,
-0.0824832618,
-0.2705151141,
-0.004103241,
0.2746977508,
0.2762577236,
-0.0568135418,
-0.0429307111,
-0.0837088376,
0.0761818588,
-0.1995842159,
-0.2112318575,
-0.3830579519,
0.1804931462,
-0.1026849002,
-0.0495566353,
-0.0805135667,
-0.0298240446,
-0.1119440049,
-0.2530979216,
-0.1765733808,
0.1710342169,
-0.1491255015,
0.3884041011,
-0.0165547654,
0.3359569013,
0.0793647543,
-0.1874969751,
-0.1123814583,
0.0434571691,
-0.0540188327,
0.3798465133,
0.063410148,
0.2255601734,
-0.2784628272,
-0.3539013863,
-0.3619383574,
0.5960182548,
0.1159186289,
-0.0575565882,
-0.3417953849,
0.1050299555,
-0.0232627857,
0.1765860617,
-0.0566633046,
0.2489113957,
-0.1592449248,
0.0920144394,
-0.4346443415,
-0.0472102985,
0.5104726553,
-0.8678762317,
-0.2605216503,
0.003188004,
0.0732160285,
-0.0388209298,
-0.2040878981,
-0.6355014443,
0.1892228127,
0.3016796112,
0.1750744879,
-0.0031793572,
0.227770552,
-0.1221882775,
-0.0525792949,
-0.0560737103,
-0.3247557878,
-0.0901469663,
-0.1945209801,
0.0034506992,
-0.2350439876
]
|
https://github.com/huggingface/datasets/issues/1973 | Question: what gets stored in the datasets cache and why is it so huge? | Echo'ing this observation: I have a few datasets in the neighborhood of 2GB CSVs uncompressed, and when I use something like `Dataset.save_to_disk()` it's ~18GB on disk.
If this is unexpected behavior, would be happy to help run debugging as needed. | I'm running several training jobs (around 10) with a relatively large dataset (3M samples). The datasets cache reached 178G and it seems really large. What is it stored in there and why is it so large? I don't think I noticed this problem before and seems to be related to the new version of the datasets library. Any insight? Thank you! | 40 | Question: what gets stored in the datasets cache and why is it so huge?
I'm running several training jobs (around 10) with a relatively large dataset (3M samples). The datasets cache reached 178G and it seems really large. What is it stored in there and why is it so large? I don't think I noticed this problem before and seems to be related to the new version of the datasets library. Any insight? Thank you!
Echo'ing this observation: I have a few datasets in the neighborhood of 2GB CSVs uncompressed, and when I use something like `Dataset.save_to_disk()` it's ~18GB on disk.
If this is unexpected behavior, would be happy to help run debugging as needed. | [
-0.0360605754,
-0.0662573799,
-0.1086275727,
0.5382815003,
0.1768521369,
0.3046649992,
-0.0687093884,
0.2563446164,
-0.1498933136,
-0.1115516722,
-0.0044143647,
-0.2384365052,
-0.1599157602,
-0.229541868,
0.1550872624,
0.2438127249,
0.1417649388,
-0.0338773765,
-0.0882984996,
-0.0986520946,
-0.1149731427,
0.0371304266,
0.0000266051,
-0.1759964228,
-0.5011150837,
-0.1951546669,
-0.1269188821,
-0.0226081572,
-0.1835440993,
-0.1439900249,
0.2084015757,
-0.0900363326,
0.3271474242,
0.4473100901,
-0.0001115048,
-0.3621959686,
0.3227519095,
-0.008409204,
-0.3896472454,
0.3312594593,
-0.3773736656,
-0.3923427761,
-0.1046534851,
-0.1323723048,
0.3790542185,
-0.0305659752,
-0.0775611848,
-0.4155648351,
-0.1001974717,
0.2361391783,
0.258138746,
-0.2053125054,
-0.3442172408,
0.1373019367,
0.1090575457,
0.073713392,
-0.157326743,
-0.2792851627,
0.2627801597,
0.2356605679,
-0.0979842022,
0.1034083962,
0.0833162889,
-0.0201201662,
0.2829734087,
-0.0790503547,
-0.3153596222,
-0.2074171603,
0.6619532704,
0.2052866071,
0.8683168292,
-0.3482805789,
-0.080823414,
-0.2358756959,
-0.1497370452,
-0.2302526683,
0.1697392315,
0.4599975646,
0.0505639613,
0.0040055164,
-0.5909304619,
-0.4143615961,
-0.1268030405,
-0.1330242157,
-0.2453346252,
-0.2898695469,
-0.3402656019,
0.0371110328,
0.1638762951,
-0.0343986861,
0.3052968085,
-0.3209095597,
-0.0971699879,
0.202012822,
-0.3501281142,
-0.2808209658,
-0.1091754138,
0.4334007502,
0.2432495654,
0.0263102837,
-0.2546925545,
-0.1597530246,
-0.1192893162,
-0.0493230931,
0.2371750325,
0.4153015018,
-0.104251124,
0.0482303798,
-0.04939726,
-0.3486228585,
-0.1449479461,
-0.1352477521,
0.1227399707,
0.0719256997,
0.472391516,
-0.4745314419,
-0.1642239988,
-0.3412801027,
0.1787271798,
0.2651434243,
0.054917641,
0.0278728418,
0.0875424519,
0.1612012684,
-0.1139690354,
0.2380319685,
-0.2866080105,
-0.1712259948,
0.0806446522,
-0.1550651491,
-0.2483568192,
-0.115296334,
-0.0712156072,
0.2489610761,
0.3250065744,
-0.2040181905,
0.2558795214,
0.0902934968,
-0.0581724197,
-0.122166045,
0.1817218214,
-0.366378963,
0.4594607055,
0.3354994953,
-0.1150589958,
0.4209492207,
-0.1799027771,
0.1078725904,
-0.211325407,
0.2094638348,
-0.417365104,
-0.4349770844,
-0.0622865595,
0.0899463594,
-0.2316665798,
0.0077564032,
-0.3615065515,
0.0903247148,
0.4243741632,
0.1884946227,
0.2217033505,
-0.1052608564,
-0.3592411876,
-0.260643959,
-0.1278336495,
0.1828976125,
-0.5495998859,
0.2316555977,
-0.0171850901,
0.2038088888,
0.0265682861,
0.3862607479,
-0.0676843077,
0.0514886156,
-0.0738905594,
-0.1387886554,
-0.0273053404,
0.0410685018,
-0.6250157952,
0.176568687,
0.3490016758,
-0.0522844493,
-0.0821780413,
0.3963995576,
0.0915794745,
-0.0462789498,
-0.1137971207,
0.2983600497,
-0.2702445686,
-0.1422474533,
-0.3710265458,
-0.1000406072,
0.0486653298,
-0.0800513998,
0.0584718138,
-0.2000577152,
-0.0648280606,
0.2171604633,
-0.0068973256,
0.143134281,
0.2213949412,
0.380055517,
-0.0400362201,
0.246272102,
0.2545774579,
0.0045566736,
-0.3890993893,
0.2957886755,
0.3068702519,
-0.6919739246,
0.1563735902,
-0.101013355,
0.1169685051,
0.1049529165,
-0.0330853909,
-0.1894967407,
-0.0202210434,
0.0810447335,
0.0512780473,
-0.1019685641,
-0.0221285596,
0.4722427726,
0.0037869907,
-0.1720621735,
-0.3683246374,
-0.2058074772,
0.0847273096,
0.0366325751,
-0.177381143,
0.0202003084,
0.0359574929,
-0.1158992276,
-0.1611274481,
0.1403148174,
0.1411767006,
-0.1652724594,
0.2010639012,
0.5026521087,
-0.009897328,
0.1133932248,
0.3529168367,
-0.0542698354,
0.1295191497,
-0.1824495643,
-0.2455495447,
0.0227385424,
-0.1056364626,
0.0891259089,
-0.0018705643,
-0.1094689369,
0.0670588091,
0.0806368068,
0.2521677017,
0.049688261,
0.1556518078,
-0.1102392748,
0.30046767,
0.3928950131,
-0.0488351732,
0.2108537257,
0.4110554159,
-0.2141381651,
0.002268027,
0.1635852605,
-0.2870115042,
-0.4692256153,
0.0958474129,
0.3619617522,
0.4408290088,
0.0798802674,
0.3315306306,
-0.1054325327,
0.2634226382,
-0.1867280155,
0.0832480341,
0.0455254465,
0.0130667808,
-0.1717015058,
0.1634774357,
-0.0028156922,
-0.0295013208,
0.2800489962,
-0.081547223,
0.2360969037,
-0.0313675553,
0.4049853086,
-0.2644425333,
-0.0564952791,
-0.0029132974,
0.3724591136,
-0.266713798,
-0.1263844073,
-0.106956996,
-0.0781470537,
0.232027173,
-0.264320612,
-0.2530310154,
0.606962204,
0.0467411466,
0.1223388016,
0.1749622673,
-0.0454662889,
-0.3047429025,
0.1147944257,
0.1704497635,
-0.3243663609,
0.2530547976,
0.2005161196,
0.1197218895,
-0.2608418763,
-0.1869264245,
-0.1394842416,
0.1243320554,
0.2161899656,
-0.3505868018,
0.0301976502,
-0.2612142861,
0.1456109732,
-0.3643141091,
-0.1169226468,
-0.0494123623,
0.1684370637,
-0.0416090824,
-0.0869181901,
0.1603960693,
-0.0047823084,
-0.2482866347,
0.0057885707,
0.2127776295,
-0.1623178124,
-0.0298234839,
0.1968927383,
-0.1227707192,
0.0286220759,
-0.1135850921,
-0.0954578146,
-0.3736052513,
-0.796487391,
0.3856807947,
0.1086681932,
-0.226092726,
0.1569163799,
0.1852317303,
-0.0288626477,
0.1390070319,
-0.7766136527,
0.278110534,
-0.2700859308,
0.1859995425,
0.0507350117,
-0.0482592694,
0.2186818868,
-0.2456575781,
0.0444294624,
-0.1558121145,
-0.336345166,
-0.2957928181,
0.1520013958,
0.5615340471,
-0.2364706099,
-0.0345993266,
0.3850360811,
0.546937108,
0.4004917145,
-0.0670358762,
-0.0679815039,
0.4184622765,
0.5475921035,
-0.3115629554,
-0.0791823342,
0.2339958549,
-0.1497089863,
-0.0996913761,
0.3620905578,
0.310739845,
-0.1633523554,
0.2869632542,
0.0401581153,
0.1326719373,
0.0942767411,
0.2241771668,
-0.3098756671,
0.1005249545,
0.1926443577,
-0.1880111098,
-0.051875107,
-0.3989800215,
-0.1218359917,
-0.0243603997,
0.0610010736,
0.2646264136,
-0.1970545501,
-0.0600720607,
-0.0649956316,
0.1443313807,
0.0027431638,
0.0161044113,
-0.0121990573,
0.2155075967,
0.2796201408,
0.268699199,
0.4034874737,
-0.2655991614,
-0.0943302363,
-0.1338838041,
-0.1440498531,
-0.0571437106,
-0.0475822575,
0.0855802819,
0.2596668005,
0.109712474,
0.1156006828,
0.3220530748,
-0.0970588923,
-0.0447798036,
0.4560163021,
-0.391461581,
-0.2944031358,
0.0297591388,
-0.1912540048,
0.2687642574,
-0.0891847536,
-0.0929315165,
-0.2434484363,
-0.0358287282,
-0.0312524401,
-0.1641992778,
0.1342912167,
0.1278090477,
-0.160503611,
0.140756771,
0.2580203116,
0.1434351951,
0.0538885891,
0.2126133293,
0.121657826,
0.2759926617,
-0.0779654682,
0.193258822,
0.0723067671,
-0.0612022057,
0.1887559593,
0.0845035166,
-0.2157592326,
-0.1994145215,
-0.2605898082,
-0.0752064288,
-0.193883568,
0.2318019122,
0.0760558173,
0.184322834,
-0.2688038945,
-0.5139296651,
0.4054386914,
0.2316488773,
-0.059795782,
0.1844307035,
-0.3162144721,
-0.0129250577,
-0.0800292268,
0.007394121,
0.619427979,
-0.2254928797,
0.3763306439,
-0.1509216279,
-0.0443960615,
0.3291867077,
-0.4975791276,
-0.0549176931,
-0.0405442193,
-0.2693859339,
-0.0064734849,
-0.0443164185,
-0.1164271906,
0.0063692247,
-0.0639231056,
0.3358241618,
-0.1385196447,
0.0161021277,
0.1028257236,
0.0630026609,
0.0982584879,
-0.1343481988,
0.0600646324,
0.2710027099,
-0.1289542168,
-0.1042522267,
-0.0486169457,
0.1003939211,
0.018198723,
0.2117584497,
-0.3484105766,
-0.1806469411,
0.0193875954,
0.3263840377,
0.000404703,
-0.1931099147,
-0.0247473605,
0.1287293732,
0.2211125195,
0.2069506198,
-0.1576767117,
0.2406376749,
-0.0560303219,
0.1770825535,
0.2221259624,
-0.1428224891,
0.1603876799,
-0.0400775522,
0.0599725842,
-0.0360326171,
0.063652724,
-0.1874226779,
-0.0689611807,
0.2709846795,
0.1704345495,
-0.0534022376,
-0.2605216503,
0.0466170609,
0.1419522166,
0.0665946677,
0.1456883699,
0.3092530668,
-0.0304542053,
0.5358057022,
-0.2437466979,
-0.444132328,
-0.0491461307,
0.0895824358,
0.4706985354,
-0.296949923,
0.2461408973,
-0.0117874546,
-0.1994107664,
-0.0052337423,
0.3188084066,
0.0672563091,
0.0313019305,
0.1030186936,
-0.2232808024,
0.2156286687,
-0.1635622531,
-0.4461264014,
0.1223970279,
-0.3968326449,
-0.2381711602,
-0.1169586107,
-0.4305742681,
-0.0959670916,
-0.20706065,
0.2224828303,
0.0288556498,
-0.4427158535,
0.1704185754,
0.4168639779,
-0.240859136,
0.1030589491,
0.1197367236,
0.2150497586,
0.0983552858,
-0.0639103353,
0.0655843988,
-0.1661858112,
-0.1151801348,
-0.0573546141,
-0.1430146545,
-0.2526409626,
0.0401960388,
0.1194193214,
0.1940990537,
-0.0846005306,
-0.1608938724,
-0.341343075,
0.0277587436,
-0.2151889801,
0.0458553731,
0.3281029761,
0.2502226233,
0.052525159,
-0.0190481525,
-0.2545651197,
0.0606616922,
0.2935043275,
0.0707681552,
0.3296490908,
0.0834347233,
0.1396348625,
0.1571824849,
-0.2573203146,
-0.4219650328,
0.187233001,
-0.1020590961,
-0.0509154983,
0.2260445505,
-0.225101307,
0.2495859563,
0.4410789013,
-0.1233843416,
0.1734841466,
-0.0809799582,
-0.1859036833,
0.0557179563,
0.148850888,
0.2688775063,
-0.1151915342,
0.0335800722,
0.4176068604,
-0.2711031139,
0.066489175,
0.1544737965,
-0.0757162273,
0.0487859771,
0.1051445156,
0.4411542416,
-0.2353550643,
0.1328930259,
0.1203705519,
0.2203481793,
-0.0414519869,
0.3915824592,
0.4607020319,
0.06688945,
0.547750473,
0.1909267008,
0.273395896,
0.4535447657,
-0.1988290101,
-0.091638796,
-0.3442141712,
-0.1206761152,
0.3691906333,
-0.111983493,
0.1148330346,
-0.0498374999,
0.2011882663,
0.1710631102,
-0.4117726088,
-0.1741052866,
-0.0463554002,
-0.318481952,
-0.0562827289,
0.4661230743,
0.0097872205,
0.1980103701,
0.3031124473,
-0.0922481194,
-0.3561850488,
0.4774301648,
-0.0334119573,
-0.2023453861,
-0.2770073414,
0.2927497327,
0.1348913163,
-0.1748968661,
-0.1015882865,
0.2509456575,
-0.2589647174,
-0.0646927729,
-0.0242526941,
0.0933385119,
0.1487420201,
0.165564701,
0.0571861006,
0.3473058045,
0.139128089,
-0.0707047656,
-0.1802368611,
0.2328952253,
-0.1752928495,
-0.1302569062,
-0.0693156421,
0.1011553109,
0.094084762,
0.3056695461,
0.0186945237,
0.2601124346,
-0.2928523123,
-0.1882196516,
0.0067084222,
-0.0192583892,
-0.2305588275,
0.2583489418,
-0.3910733759,
-0.1737107933,
0.6758270264,
-0.258058995,
0.1359241158,
-0.1140785515,
0.0960623771,
-0.1910626143,
0.4656356871,
0.4458969235,
-0.0579665639,
-0.2991361618,
0.0074319211,
-0.2848970294,
0.1120930091,
-0.260648787,
0.4734935462,
-0.1006663144,
0.5078718066,
-0.1443119496,
-0.0784378424,
-0.109480992,
-0.033502508,
-0.056261722,
-0.0785239488,
-0.2845357358,
-0.0505348295,
0.114025645,
0.1673986912,
0.056568753,
-0.1680806577,
0.2948610187,
-0.2146658152,
-0.0311390273,
-0.088876538,
0.0323961861,
0.0776208341,
0.3440175951,
0.0808251798,
0.0452023856,
0.0137081416,
0.1630227864,
0.1854194999,
-0.3548761904,
0.0036778955,
0.1407096833,
0.0632229447,
0.0539238416,
-0.0235883221,
-0.519605875,
0.1973179132,
0.1435052752,
0.0603236519,
-0.1435729116,
-0.0266824793,
0.0854972675,
-0.3765103221,
-0.2722698748,
0.3478080332,
0.4459002316,
0.1441463232,
-0.1681192666,
-0.0506998748,
-0.2086983919,
-0.0880707726,
0.2826549709,
0.0581455305,
-0.3323768079,
-0.0007813748,
0.1203754097,
0.5062116981,
-0.1627059281,
-0.3356367946,
0.1049753428,
0.4289160073,
-0.1710665673,
-0.2342908084,
0.2778823376,
-0.2623939514,
0.1653280854,
-0.1241286099,
0.6173256636,
0.000172947,
-0.0431100242,
0.2192086875,
-0.1861627102
]
|
https://github.com/huggingface/datasets/issues/1973 | Question: what gets stored in the datasets cache and why is it so huge? | Thanks @ioana-blue for pointing out this problem (and thanks also @justin-yan). You are right that current implementation of the datasets caching files take too much memory. We are definitely changing this and optimizing the defaults, so that the file sizes are considerably reduced. I will come back to you as soon as this is fixed. | I'm running several training jobs (around 10) with a relatively large dataset (3M samples). The datasets cache reached 178G and it seems really large. What is it stored in there and why is it so large? I don't think I noticed this problem before and seems to be related to the new version of the datasets library. Any insight? Thank you! | 55 | Question: what gets stored in the datasets cache and why is it so huge?
I'm running several training jobs (around 10) with a relatively large dataset (3M samples). The datasets cache reached 178G and it seems really large. What is it stored in there and why is it so large? I don't think I noticed this problem before and seems to be related to the new version of the datasets library. Any insight? Thank you!
Thanks @ioana-blue for pointing out this problem (and thanks also @justin-yan). You are right that current implementation of the datasets caching files take too much memory. We are definitely changing this and optimizing the defaults, so that the file sizes are considerably reduced. I will come back to you as soon as this is fixed. | [
-0.0833512694,
0.0121253347,
-0.129175663,
0.5186684132,
0.1493166834,
0.2426840067,
-0.0757898465,
0.3019646108,
-0.0877330229,
-0.0359355286,
-0.0295593664,
-0.2534342408,
-0.0936840177,
-0.2008034289,
0.0723078474,
0.1365635842,
0.0901817903,
-0.0653724819,
-0.0765936598,
-0.0797737613,
-0.1062292084,
-0.0433258377,
-0.0135118598,
-0.1718013287,
-0.5766088963,
-0.1823078245,
-0.1253970861,
-0.0051542921,
-0.2007427067,
-0.1783480346,
0.1808401197,
-0.0291625522,
0.3237578571,
0.3823102415,
-0.000108076,
-0.3183885515,
0.35694924,
0.0258179791,
-0.3599813879,
0.3442701697,
-0.5000376701,
-0.2974620759,
-0.1312580854,
-0.1360440403,
0.3404592276,
-0.0457876995,
-0.0374124311,
-0.3942117393,
-0.0077166064,
0.2449935079,
0.2759655118,
-0.2257376462,
-0.3055835664,
0.0927580521,
0.2078103423,
0.0236136653,
-0.1338834912,
-0.2668148875,
0.2703863084,
0.1654696316,
-0.1730727404,
0.1816092283,
0.090152666,
-0.0411953628,
0.2849492133,
-0.0867874995,
-0.2421436906,
-0.2034813911,
0.6005623937,
0.2197856009,
0.9331115484,
-0.3052318394,
-0.100264363,
-0.167825833,
-0.1438966095,
-0.1512908041,
0.2134749293,
0.4073271453,
0.0708833411,
-0.0055506006,
-0.5161411762,
-0.4197383225,
-0.1441657245,
-0.1212615818,
-0.1735286415,
-0.2512040436,
-0.2740027308,
0.0589072406,
0.1617283076,
-0.0618542619,
0.4042037427,
-0.2412801981,
-0.1609717011,
0.1731909513,
-0.3817915618,
-0.2755790353,
-0.2229239792,
0.4864883423,
0.2402956039,
0.0038445157,
-0.2600578368,
-0.1092920676,
-0.1039827392,
-0.0202955194,
0.2225334644,
0.3948452175,
-0.1059476659,
0.1032146439,
-0.0339700207,
-0.3564938307,
-0.2345283628,
-0.1782754213,
0.1071282774,
0.0232658219,
0.4347734749,
-0.4517469108,
-0.1963359565,
-0.3539863825,
0.2049480379,
0.1451402903,
-0.0209947079,
-0.0245745853,
0.1459488124,
0.1945824176,
-0.1634192169,
0.2311791927,
-0.291672945,
-0.2418981791,
0.0145780277,
-0.2142632455,
-0.2492373288,
-0.062359456,
-0.1481208354,
0.3357028067,
0.3038077354,
-0.1790137589,
0.28062433,
0.0677448139,
-0.0248507615,
-0.142868802,
0.2385396957,
-0.3573530912,
0.4010713995,
0.3282658458,
-0.1311091036,
0.2729567587,
-0.2001667917,
0.1455440223,
-0.1907134652,
0.1966865361,
-0.5129279494,
-0.454575181,
-0.0051716524,
0.1176293641,
-0.1054759026,
0.037894059,
-0.3192513585,
0.0472881086,
0.413082093,
0.1691479683,
0.1751929075,
-0.1207907796,
-0.4018247724,
-0.2324275076,
-0.1263592988,
0.1870443523,
-0.3892208636,
0.2134617269,
0.0099466173,
0.2114599198,
0.0577603728,
0.383623153,
-0.1483820826,
0.0776752383,
-0.0870076194,
-0.1209376156,
0.000155843,
-0.0795793906,
-0.6367112994,
0.1218228191,
0.3812288046,
-0.1184330955,
-0.0189937018,
0.4304908812,
0.0283242762,
-0.0962786451,
-0.1710999161,
0.2358876765,
-0.2705535591,
-0.1395440996,
-0.2917769551,
-0.1208133847,
-0.0083787851,
-0.0380459242,
0.0865778923,
-0.1643363535,
-0.014614664,
0.3307115138,
-0.0058595696,
0.1374899745,
0.086740382,
0.3432824612,
-0.0301415697,
0.261325568,
0.3053283989,
0.0460472442,
-0.4341361523,
0.2702081203,
0.1865425706,
-0.6862899661,
0.1906160861,
-0.1346432716,
0.1436362565,
0.0420824513,
-0.0126285534,
-0.1946733594,
0.020759752,
0.0416757353,
0.0679129958,
-0.2097645849,
-0.0188924056,
0.5739926696,
-0.0988555178,
-0.1863345951,
-0.3965401351,
-0.2163491696,
0.0431044884,
0.0391311273,
-0.0731344372,
-0.0137702813,
0.0558139682,
-0.089211002,
-0.1688680053,
0.1361526102,
0.2321186066,
-0.1308442801,
0.2174190134,
0.5695485473,
-0.0355298035,
0.0977272987,
0.3712706268,
-0.1007414907,
0.0756177083,
-0.1984052956,
-0.2419497669,
-0.0179809146,
-0.1506019831,
0.1063495651,
0.02781781,
-0.1154015288,
0.0016546703,
0.0606406815,
0.2964202762,
0.0728687793,
0.1380551606,
-0.1058860198,
0.3245922327,
0.4382674992,
-0.0780997202,
0.176500231,
0.4605900645,
-0.1509391963,
-0.0410192274,
0.1564589739,
-0.2423934788,
-0.47892946,
0.1506143361,
0.3311380446,
0.3907627761,
0.1092209965,
0.2850664258,
-0.1033122465,
0.2868414819,
-0.160823971,
0.0812225342,
0.0667130202,
0.0219489764,
-0.2884733379,
0.0932127461,
-0.0410420708,
-0.0126916394,
0.2164717466,
-0.0556183383,
0.2983462512,
-0.0109922839,
0.3491844833,
-0.2550542653,
-0.1621958613,
-0.0070125479,
0.3860312402,
-0.2329478115,
-0.1407173127,
-0.1002913713,
-0.0584417358,
0.2660433352,
-0.1486243159,
-0.2300077081,
0.5778571963,
0.0820519924,
0.1423573345,
0.0880350471,
-0.0799467266,
-0.3060518503,
0.111075215,
0.2493349463,
-0.3045134842,
0.1999037862,
0.1163127869,
0.0619492568,
-0.2768293917,
-0.1332399398,
-0.1086861491,
0.1835202724,
0.1987149119,
-0.302762419,
0.0109499423,
-0.3178111315,
0.1380323172,
-0.3626976013,
-0.1848440915,
-0.1165109724,
0.1484915912,
-0.0446067825,
-0.00623322,
0.2158528417,
-0.0736986846,
-0.162884593,
-0.0196523909,
0.1723953933,
-0.1615502685,
0.0438600443,
0.3077872992,
-0.1309023201,
0.0314122029,
-0.2782613039,
-0.0471065529,
-0.3229494989,
-0.8187433481,
0.2975212634,
0.1009914353,
-0.2260670811,
0.1321330369,
0.2460593134,
0.0601856038,
0.1901033819,
-0.7528632879,
0.1599376798,
-0.2401581854,
0.1551106274,
-0.001820003,
-0.0307545438,
0.2011797875,
-0.1798160523,
0.0073360559,
-0.1245790869,
-0.196788311,
-0.2765602171,
0.1456561238,
0.4915039539,
-0.192502901,
-0.0633853003,
0.3370989263,
0.5671010017,
0.5190479755,
0.0446702875,
-0.070525825,
0.3782271445,
0.4487401843,
-0.3152043521,
-0.0880659968,
0.2686747909,
-0.1435837299,
-0.0421168283,
0.4030549824,
0.3427501917,
-0.1661659181,
0.2124954611,
-0.0696431622,
0.1701493859,
0.0360479727,
0.1999655366,
-0.2818180025,
0.1630782336,
0.1915143877,
-0.2135234028,
-0.0805289447,
-0.409075141,
-0.0097259274,
-0.0490242094,
0.0604404733,
0.3139722943,
-0.2041912675,
-0.0216280464,
-0.0354798883,
0.0954541862,
0.0373732224,
0.0340193361,
-0.0738481432,
0.2846225798,
0.2186450064,
0.3076268733,
0.3758858144,
-0.1845826805,
-0.1280830204,
-0.194876954,
-0.1542896181,
0.049772352,
0.0045884242,
0.0770997331,
0.3125368059,
0.1059293151,
0.120621711,
0.3195888102,
-0.1694953293,
-0.063276127,
0.4400777817,
-0.3505928814,
-0.3430589139,
0.0311940238,
-0.1552644372,
0.2621398866,
-0.1166705415,
-0.0711958855,
-0.2251217812,
0.0282575935,
-0.0399835482,
-0.1176181957,
0.0885974392,
0.1425408423,
-0.1457243264,
0.1115197167,
0.2330705076,
0.11776115,
0.1649421453,
0.1629831195,
0.1325432509,
0.2992494702,
-0.10561046,
0.1794901639,
0.0435791202,
-0.0517015941,
0.190840885,
0.0886831433,
-0.2106286883,
-0.19950369,
-0.217627719,
-0.104385443,
-0.1774016768,
0.2469910383,
0.0499367118,
0.1593246311,
-0.2842365205,
-0.5892305374,
0.4678242803,
0.2875882685,
-0.05030334,
0.2816500366,
-0.3000888824,
-0.0262129325,
-0.1170728281,
0.0719797015,
0.6399997473,
-0.217084989,
0.3547423482,
-0.160719797,
-0.0057512769,
0.325263232,
-0.4948310256,
-0.0203317534,
-0.0317215994,
-0.2943031192,
0.0027604913,
-0.0793969259,
-0.1042395234,
0.0160080232,
-0.0375991426,
0.3806455731,
-0.0770794824,
0.0171459038,
0.1023031101,
0.2111572027,
0.0683916211,
-0.1219476312,
0.1412032545,
0.2821707726,
-0.1376041323,
-0.1486558169,
-0.0250101909,
0.1032503173,
0.0334871933,
0.2228504419,
-0.2927022576,
-0.1754135191,
-0.0282165054,
0.3199632764,
-0.0239456072,
-0.1103062555,
0.0224185325,
0.150846228,
0.1966028512,
0.2202225477,
-0.1755134314,
0.2917010486,
-0.0590801686,
0.1441818625,
0.2262177914,
-0.1373252124,
0.180284217,
-0.092255339,
-0.0155959539,
-0.0061956984,
0.0756168216,
-0.207546398,
-0.1815807223,
0.259036243,
0.1753004342,
-0.0318701789,
-0.1809736937,
0.0111451196,
0.0968551859,
0.0720029026,
0.1782183498,
0.3341041505,
-0.0147101516,
0.5450963974,
-0.1917357594,
-0.4085084796,
-0.0390248932,
0.0376458019,
0.4952646494,
-0.2649908066,
0.296145618,
0.0108361887,
-0.2105103582,
-0.0300778877,
0.2401516885,
0.1065252349,
-0.0192215722,
0.0464050695,
-0.1987548918,
0.2384640723,
-0.1002333537,
-0.3699207604,
0.0672204047,
-0.3693827987,
-0.1530646384,
-0.1152308211,
-0.473905623,
-0.0556581989,
-0.2485063076,
0.217540592,
-0.0033827799,
-0.4405686259,
0.1212479025,
0.4964891672,
-0.2847179472,
0.0173553471,
0.0766953081,
0.2561144829,
0.0128195593,
-0.1073093861,
0.0661791265,
-0.1396747082,
-0.0889872089,
-0.0818246752,
-0.1462653875,
-0.2698168457,
0.064040795,
0.1060322002,
0.1788945645,
-0.0970168635,
-0.1527719349,
-0.3858825564,
-0.0040501161,
-0.2405211329,
0.0508170575,
0.2874115109,
0.2743218839,
0.0970946252,
0.0281570498,
-0.3459438086,
0.1409606934,
0.3457018137,
0.0663543269,
0.2746599019,
0.057415884,
0.1722719073,
0.1525082588,
-0.2675608397,
-0.3174440563,
0.1916095465,
-0.10968633,
-0.1107755005,
0.1850316823,
-0.2056340873,
0.2195074856,
0.4261026382,
-0.1534728557,
0.1396077871,
-0.0626813248,
-0.1825830787,
0.1420996189,
0.1769848168,
0.2107806802,
-0.155299589,
0.0270277057,
0.3825100064,
-0.2631723881,
0.1017865837,
0.1827222109,
-0.0995819643,
0.1150414199,
0.0916785002,
0.3488770425,
-0.3227350712,
0.0893633664,
-0.0018472678,
0.2343329936,
-0.1470897347,
0.4342316985,
0.5094914436,
0.0556075014,
0.5676191449,
0.2129420042,
0.3438633084,
0.4268431962,
-0.135420993,
-0.0707474649,
-0.2690297067,
-0.2201738358,
0.431825012,
-0.1616874486,
0.0600058846,
-0.09151043,
0.2003552318,
0.2525607944,
-0.3557161391,
-0.1063615307,
-0.0910750702,
-0.2840984464,
-0.0544140376,
0.4328333139,
0.0195153523,
0.16266267,
0.2804258168,
-0.0502699874,
-0.3196660876,
0.4784550965,
0.0176237896,
-0.2315434813,
-0.2045262456,
0.2657096684,
0.2216134518,
-0.0962503254,
-0.150314942,
0.2840473652,
-0.2878264487,
-0.0521468185,
-0.0020573011,
0.1353497058,
0.1079745889,
0.1173432693,
0.0900893807,
0.2902496457,
0.1260781437,
-0.1177471876,
-0.1577918977,
0.2456448525,
-0.1479883492,
-0.1631239653,
-0.0584238172,
0.1051508263,
0.0860579386,
0.1809344143,
-0.0109625617,
0.2041433305,
-0.2903202474,
-0.1878156513,
0.0826531425,
-0.0291631259,
-0.1981458515,
0.3062012494,
-0.398968935,
-0.1746678799,
0.6400589943,
-0.2770073116,
0.0793249905,
-0.1532568783,
0.1183214784,
-0.2559441924,
0.5393369198,
0.4638427496,
0.0643789023,
-0.2885503471,
-0.066926755,
-0.218408674,
0.0996607542,
-0.3270800114,
0.4932590127,
-0.0297251604,
0.469866991,
-0.2054981738,
-0.1049917191,
-0.0908685923,
-0.1021396816,
-0.0105251083,
-0.1008917466,
-0.3023268878,
-0.0487519205,
0.1350870132,
0.1893382967,
0.0312540047,
-0.1479460299,
0.3177110851,
-0.2440876812,
0.0139771793,
-0.1394677907,
-0.0066866288,
0.1513635069,
0.355455786,
0.0827658027,
0.0488253161,
-0.0478212424,
0.1564612538,
0.1493870914,
-0.3969496489,
-0.0511360914,
0.085100472,
-0.0666461214,
0.0280112848,
-0.0466873981,
-0.5293644071,
0.1965585351,
0.0999142304,
0.1286890805,
-0.2723075151,
-0.0669397414,
0.0615589283,
-0.3856152296,
-0.1979496181,
0.3814426363,
0.3347819448,
0.1350271106,
-0.1180507019,
-0.1002390981,
-0.2879347503,
-0.0645389035,
0.2868933976,
0.0080704624,
-0.3462716341,
0.0657005012,
0.1039325893,
0.4476659,
-0.1230792478,
-0.3959045708,
0.1256317049,
0.4089437723,
-0.0978844315,
-0.1693585962,
0.227812171,
-0.1347614974,
0.2264449745,
-0.0989769921,
0.5771960616,
-0.0549584217,
-0.0072085382,
0.2037746459,
-0.1564335972
]
|
https://github.com/huggingface/datasets/issues/1973 | Question: what gets stored in the datasets cache and why is it so huge? | Thank you! Also I noticed that the files don't seem to be cleaned after the jobs finish. Last night I had only 3 jobs running, but the cache was still at 180GB. | I'm running several training jobs (around 10) with a relatively large dataset (3M samples). The datasets cache reached 178G and it seems really large. What is it stored in there and why is it so large? I don't think I noticed this problem before and seems to be related to the new version of the datasets library. Any insight? Thank you! | 32 | Question: what gets stored in the datasets cache and why is it so huge?
I'm running several training jobs (around 10) with a relatively large dataset (3M samples). The datasets cache reached 178G and it seems really large. What is it stored in there and why is it so large? I don't think I noticed this problem before and seems to be related to the new version of the datasets library. Any insight? Thank you!
Thank you! Also I noticed that the files don't seem to be cleaned after the jobs finish. Last night I had only 3 jobs running, but the cache was still at 180GB. | [
-0.1613564193,
0.0733158514,
-0.1333291233,
0.5806112289,
0.0784173682,
0.2742607892,
-0.0560066402,
0.245710358,
-0.124497965,
-0.0830652267,
-0.0408940762,
-0.2797769606,
-0.1030298099,
-0.1785739958,
0.0967971906,
0.2181342095,
0.0879471526,
-0.120729737,
-0.1814739406,
-0.1622635722,
-0.1023326218,
-0.0179167055,
0.0224895552,
-0.1180054918,
-0.6523389816,
-0.2212353349,
-0.1131067872,
0.0551730022,
-0.1404676735,
-0.0933791324,
0.1439766884,
-0.0460862108,
0.3950193822,
0.5104983449,
-0.0001139643,
-0.2925598323,
0.3823722303,
0.0545069762,
-0.4045554698,
0.3270022571,
-0.4570841193,
-0.3279615045,
-0.1085925922,
-0.1003949717,
0.4394217432,
-0.0204238556,
0.0014368225,
-0.3428093493,
-0.018662909,
0.2640453875,
0.2358754873,
-0.3048944771,
-0.3635191917,
0.1135003492,
0.1681372374,
0.1231584847,
-0.0441246442,
-0.2613188624,
0.2455048114,
0.1298616529,
-0.1326352805,
0.1569847912,
0.1074743196,
-0.0296068564,
0.1850610673,
-0.0502868295,
-0.216455698,
-0.2998596728,
0.7091449499,
0.2274082899,
0.8417987823,
-0.2384048998,
-0.0828320011,
-0.2749612629,
-0.1479181647,
-0.1580326259,
0.220784843,
0.5174490213,
0.1128785908,
-0.0317041799,
-0.511755228,
-0.3802758455,
-0.0655807108,
-0.1840290874,
-0.1793048829,
-0.2046904117,
-0.3094809353,
0.0825136974,
0.148161158,
-0.0059113558,
0.3916047215,
-0.2668058872,
-0.1414569765,
0.2316582948,
-0.3380457163,
-0.248155266,
-0.1725747138,
0.5365597606,
0.1817599386,
-0.0329652913,
-0.3444200456,
-0.1124418974,
-0.211458981,
-0.0000930539,
0.2603338063,
0.3116799295,
-0.0861700401,
0.1187399179,
0.0085731633,
-0.385429889,
-0.3219988346,
-0.1497399956,
0.0372535661,
0.022133546,
0.3994229734,
-0.4164707065,
-0.1734294295,
-0.3614161015,
0.1985379905,
0.1956635416,
-0.0042333603,
-0.0722751841,
0.1121164337,
0.2183163315,
-0.1871270239,
0.2036715448,
-0.2611679137,
-0.2313588411,
0.0284114853,
-0.153280586,
-0.228550896,
-0.0909062028,
-0.1327640265,
0.2995838225,
0.2765157819,
-0.139654845,
0.3309640884,
0.0368877538,
-0.0648177192,
-0.1563340276,
0.2549434006,
-0.3582816124,
0.3951001167,
0.4093770087,
-0.1124285012,
0.3327394426,
-0.2217198312,
0.2115443945,
-0.1827075034,
0.2137182206,
-0.5258375406,
-0.44183442,
-0.0159433987,
0.0983832777,
-0.1572526842,
0.0768249333,
-0.3453715444,
-0.0189489294,
0.4571040571,
0.2275616229,
0.2256824672,
-0.164601624,
-0.3279083967,
-0.1894808114,
-0.2278370112,
0.1464493126,
-0.391038239,
0.2511364222,
0.0179404989,
0.1454427242,
0.0711841211,
0.2979474366,
-0.1191539317,
0.1315391809,
-0.0978695154,
-0.1348293424,
0.0279833209,
-0.0406081527,
-0.5370237231,
0.1421535164,
0.400799334,
-0.1622821987,
-0.0745797753,
0.3459578753,
0.029050529,
-0.067992866,
-0.1514484435,
0.2860537469,
-0.2108335346,
-0.1279176474,
-0.2654141486,
-0.0885378867,
-0.0383824818,
-0.0184706636,
0.0998506993,
-0.2232893854,
-0.0368052758,
0.1881958991,
0.028792806,
0.1677854955,
0.0976626426,
0.3306000233,
-0.1397836953,
0.4280256331,
0.2879229188,
0.0306460727,
-0.4317773283,
0.2343403548,
0.1349746138,
-0.6966280937,
0.1623963416,
-0.1269114614,
0.2554292679,
0.0729259327,
-0.0731002167,
-0.2337892205,
-0.0279713571,
0.1845548004,
0.0301330611,
-0.1857676506,
-0.0711146668,
0.4955204129,
-0.1140736341,
-0.1607789844,
-0.429720968,
-0.1848447621,
0.1431547999,
-0.002323675,
-0.1459567845,
0.0411186144,
0.0786861256,
-0.1117614135,
-0.1637638062,
0.1110551581,
0.2477664202,
-0.1484707445,
0.2682820261,
0.5673515797,
-0.0548565686,
0.195150435,
0.3002673388,
-0.0716318265,
0.0636251271,
-0.17340675,
-0.2402175367,
-0.0508689284,
-0.2077073902,
0.1525836736,
0.0015327329,
-0.1117343381,
0.0542668886,
0.0190220959,
0.2636957467,
0.1293397099,
0.1632872373,
-0.0756036565,
0.2168035358,
0.444778353,
-0.1519054323,
0.1747808754,
0.4706735611,
-0.1771166176,
-0.0516840965,
0.1113191396,
-0.2850254476,
-0.4193942547,
0.1050441712,
0.4016010165,
0.4452231824,
0.0496108979,
0.3604387939,
-0.0756825879,
0.277546674,
-0.1837284416,
0.0208873451,
0.074898988,
-0.0487935655,
-0.1522930264,
0.0795727298,
-0.007895479,
-0.0303096753,
0.1681204289,
-0.0738716647,
0.290492028,
-0.0363349356,
0.357268393,
-0.2580594122,
-0.1316239685,
-0.0732910708,
0.3482123911,
-0.2150788307,
-0.1365127861,
-0.1094917431,
-0.070986338,
0.2380698919,
-0.1624611169,
-0.2139177024,
0.5364331603,
-0.0142113958,
0.1500573903,
0.073552385,
-0.089329496,
-0.3087902367,
0.0690323412,
0.2572315633,
-0.2098606825,
0.2047889531,
0.0922111496,
0.066483818,
-0.2757597566,
-0.1237824038,
-0.1766272336,
0.195168376,
0.2061214894,
-0.2882382274,
0.0006669185,
-0.2626595497,
0.0905258209,
-0.3399088383,
-0.1441927105,
-0.0432295911,
0.0609960705,
-0.0696480796,
0.0072278962,
0.2078795284,
-0.0054570534,
-0.2065434903,
-0.0260301381,
0.1936480552,
-0.1558230221,
-0.0068774233,
0.2063535005,
-0.2218585163,
0.0161887463,
-0.2453484833,
-0.0151543487,
-0.3820663989,
-0.9283381701,
0.259250164,
0.0368521921,
-0.1697474718,
0.2085365057,
0.1965107918,
0.0232021529,
0.1615057439,
-0.7610758543,
0.2020998299,
-0.2372549772,
0.1262796968,
0.007772943,
0.0311024114,
0.2027198374,
-0.1744493842,
0.0423538014,
-0.1398318559,
-0.2366736382,
-0.3054130673,
0.1870910227,
0.5237815976,
-0.2106374204,
-0.0840957686,
0.1811248064,
0.536593616,
0.4866471291,
-0.0381649248,
-0.1205075085,
0.4225434363,
0.4848381877,
-0.2912489176,
-0.078384459,
0.2911514938,
-0.0866433904,
-0.0904414356,
0.4184951186,
0.3539145291,
-0.0811956376,
0.2511944771,
-0.0332705043,
0.040465001,
0.0854172036,
0.2601004839,
-0.3480749726,
0.1371674836,
0.1285502166,
-0.1664552838,
-0.0566878468,
-0.3869127631,
-0.0410663895,
-0.008741986,
0.0842421651,
0.2667951584,
-0.1963015497,
-0.093753837,
0.0104852952,
0.1013093367,
-0.0088180536,
-0.0358110964,
-0.06187886,
0.29164657,
0.2779176235,
0.3221158385,
0.3650981784,
-0.3677512109,
-0.0626020133,
-0.1375657022,
-0.1767584085,
0.059802644,
-0.0522745959,
0.03419194,
0.2674120665,
0.1784341633,
0.1854331046,
0.3122754097,
-0.1331875324,
0.0565019734,
0.4340999722,
-0.3935497403,
-0.3147127032,
0.0180362798,
-0.1934535503,
0.2668856382,
-0.0967533141,
-0.113451913,
-0.2543799579,
-0.0134459995,
-0.0458250828,
-0.1563430727,
0.1244401783,
0.1552250832,
-0.1609626114,
0.0811807513,
0.3141673803,
0.111978285,
0.1702610254,
0.2174152881,
0.1782796383,
0.3218364716,
-0.1787976623,
0.2074741721,
0.0910575092,
-0.0685986653,
0.2785597742,
0.0779270977,
-0.1736047566,
-0.2642303407,
-0.2368405759,
-0.0679097623,
-0.1778142452,
0.274741441,
0.0785837248,
0.2560665905,
-0.2771653533,
-0.5995444655,
0.3857508898,
0.2862665951,
-0.1092851534,
0.2671399117,
-0.489554137,
-0.0106043257,
-0.1321154088,
-0.0013051231,
0.6466197371,
-0.2087158114,
0.3260279,
-0.199916169,
-0.0667582527,
0.3643880188,
-0.5225835443,
-0.0769260898,
-0.0006266074,
-0.203223303,
-0.0374313779,
-0.0765036419,
-0.1397908032,
0.0380021706,
-0.0022656065,
0.3384397328,
-0.0816518739,
0.0173255894,
0.0731510594,
0.1452866942,
0.0633621216,
-0.0883146077,
0.117008239,
0.2121533453,
-0.1198507994,
-0.0831394047,
-0.0899271592,
0.1223375425,
0.1065190583,
0.2631841302,
-0.2684571147,
-0.1861672699,
-0.016653534,
0.2970584929,
0.0237219539,
-0.1567719579,
-0.0804013237,
0.193159014,
0.1869922578,
0.1478960663,
-0.1096017659,
0.2880506814,
-0.0981836841,
0.1249378547,
0.194135651,
-0.1072759479,
0.2267088443,
-0.0649361387,
-0.0248343367,
0.0245962832,
0.0448239259,
-0.1732370257,
-0.2194975019,
0.3592064977,
0.1631357521,
-0.0518305749,
-0.2033404857,
0.0533612482,
0.0576287881,
0.1359995902,
0.1384759992,
0.3363604248,
-0.0736915618,
0.6109345555,
-0.217841804,
-0.4782263637,
-0.0767514408,
-0.0232028887,
0.503282845,
-0.2337807566,
0.2860403061,
0.0184661523,
-0.1751321107,
-0.0311499927,
0.255908072,
0.1470766664,
0.0428167842,
0.0856798366,
-0.1649221778,
0.2176070809,
-0.0642014593,
-0.4341560602,
0.0132349264,
-0.3945585191,
-0.2324021757,
-0.1734167039,
-0.435887903,
-0.0303707533,
-0.2216002047,
0.2527506351,
0.0294939522,
-0.3938134015,
0.2168480605,
0.4286862314,
-0.2361280471,
0.1436594129,
0.0652047396,
0.3111397922,
0.06429068,
-0.0576628372,
0.0879252553,
-0.1032583416,
-0.1550846696,
-0.0653668568,
-0.1248484328,
-0.2228999734,
0.0525919087,
0.1372590512,
0.1883081645,
-0.0488750599,
-0.1431195438,
-0.3217912018,
0.0851672813,
-0.2121694684,
0.0646224543,
0.18694444,
0.2090810835,
0.156413272,
0.1074779779,
-0.2736563087,
0.0798653215,
0.3291402161,
0.0449233912,
0.2957023382,
0.0472167097,
0.1226036027,
0.1379896849,
-0.2660233974,
-0.3409767449,
0.0906478539,
-0.0949323326,
-0.0699299946,
0.2095037699,
-0.2170517594,
0.2317304909,
0.432890296,
-0.126177907,
0.2592009008,
-0.0209148992,
-0.1793332994,
0.179448992,
0.1302596778,
0.2233305126,
-0.0603437126,
0.0664516911,
0.4383388162,
-0.3042236567,
0.0979854167,
0.1023674458,
-0.1207405999,
0.0606675446,
0.1509357542,
0.3464744389,
-0.3671174943,
0.117305547,
0.0725597739,
0.1537766755,
-0.0700300261,
0.4517394006,
0.4793986976,
0.0183851682,
0.5429627299,
0.3200547695,
0.4149708152,
0.4656386673,
-0.1855344325,
-0.0896624923,
-0.3549956977,
-0.2353119254,
0.5174937248,
-0.1213667989,
0.0425862186,
-0.1281031519,
0.1910884529,
0.2133020461,
-0.3449544609,
-0.1182984933,
-0.0223556161,
-0.2834680378,
-0.0617319793,
0.3831986487,
0.0348753296,
0.2570714355,
0.3279586732,
-0.0391799584,
-0.2646866441,
0.5557456017,
-0.0171337072,
-0.2771168947,
-0.2427121997,
0.2536898255,
0.1225281432,
-0.1367987543,
-0.1073720157,
0.2526152134,
-0.3126507998,
-0.0337106884,
-0.0128570581,
0.1473042667,
0.1936822385,
0.1900820732,
-0.0141223827,
0.3107691407,
0.0938917473,
-0.0519732423,
-0.2107859999,
0.336796701,
-0.1680718064,
-0.1389776766,
-0.0691706017,
0.09623193,
0.0966415778,
0.2490072399,
-0.0509269722,
0.223356396,
-0.230883643,
-0.1931045651,
0.1338281184,
-0.0147660458,
-0.2211968452,
0.2575014532,
-0.3979862928,
-0.1736106426,
0.6114830971,
-0.2598449886,
0.0722600892,
-0.1495043188,
0.0701110065,
-0.2653841674,
0.5645395517,
0.5225408077,
0.0885150433,
-0.2780854404,
-0.0863829479,
-0.2743104994,
0.0643814504,
-0.3352907002,
0.5271923542,
-0.1185420901,
0.4859296679,
-0.2018981725,
-0.1149546206,
-0.0428571887,
-0.1220419928,
-0.0005124023,
-0.1198475137,
-0.3422718346,
-0.0923208892,
0.1259982288,
0.1910202652,
0.0899186805,
-0.1604305804,
0.2859536409,
-0.23846367,
-0.0576169752,
-0.053892903,
-0.0453091748,
0.1033480987,
0.3779554963,
0.146268338,
0.0711489171,
-0.0091990698,
0.1375866234,
0.2291774154,
-0.3952299953,
-0.0751998127,
0.1247664019,
0.0192074031,
0.0290724374,
-0.0575016513,
-0.567225039,
0.2911174893,
0.1137890741,
0.0580926016,
-0.2475100011,
-0.0398725457,
0.092694968,
-0.4392721057,
-0.1869779527,
0.3382077515,
0.3680441082,
0.1801198721,
-0.1165713295,
-0.1416971534,
-0.241491884,
-0.0349173136,
0.3328413069,
0.0415234715,
-0.4040129781,
-0.0493650548,
0.0916973948,
0.415422976,
-0.0620094612,
-0.41349262,
0.1519258618,
0.4260598421,
-0.0775111318,
-0.1994902641,
0.1650744379,
-0.0829911903,
0.2037354112,
-0.0660083666,
0.4899358749,
-0.0626695454,
-0.0396935195,
0.2204193175,
-0.1378958821
]
|
https://github.com/huggingface/datasets/issues/1973 | Question: what gets stored in the datasets cache and why is it so huge? | Hi ! As Albert said they can sometimes take more space that expected but we'll fix that soon.
Also, to give more details about caching: computations on a dataset are cached by default so that you don't have to recompute them the next time you run them.
So by default the cache files stay on your disk when you job is finished (so that if you re-execute it, it will be reloaded from the cache).
Feel free to clear your cache after your job has finished, or disable caching using
```python
import datasets
datasets.set_caching_enabled(False)
``` | I'm running several training jobs (around 10) with a relatively large dataset (3M samples). The datasets cache reached 178G and it seems really large. What is it stored in there and why is it so large? I don't think I noticed this problem before and seems to be related to the new version of the datasets library. Any insight? Thank you! | 95 | Question: what gets stored in the datasets cache and why is it so huge?
I'm running several training jobs (around 10) with a relatively large dataset (3M samples). The datasets cache reached 178G and it seems really large. What is it stored in there and why is it so large? I don't think I noticed this problem before and seems to be related to the new version of the datasets library. Any insight? Thank you!
Hi ! As Albert said they can sometimes take more space that expected but we'll fix that soon.
Also, to give more details about caching: computations on a dataset are cached by default so that you don't have to recompute them the next time you run them.
So by default the cache files stay on your disk when you job is finished (so that if you re-execute it, it will be reloaded from the cache).
Feel free to clear your cache after your job has finished, or disable caching using
```python
import datasets
datasets.set_caching_enabled(False)
``` | [
-0.0611621961,
-0.0334957354,
-0.1297228783,
0.512793839,
0.1173945814,
0.2473039925,
-0.0104232961,
0.2427642494,
-0.0756754428,
-0.0384479873,
-0.0576454923,
-0.2856125236,
-0.0628410876,
-0.1385335475,
0.1360729635,
0.0736557618,
0.0571288578,
-0.0924019367,
-0.0630483255,
-0.1605250239,
-0.1804127991,
0.0023385545,
-0.0435125344,
-0.1090049148,
-0.606941402,
-0.1671301723,
-0.1760504246,
0.0133184446,
-0.1266204864,
-0.2256068736,
0.1812642813,
-0.0810928568,
0.3298203945,
0.5128856897,
-0.0001129724,
-0.3193744719,
0.3587916493,
0.0623998903,
-0.4379306138,
0.2950620949,
-0.3354636133,
-0.427118808,
-0.0203633644,
-0.1686972827,
0.321441263,
-0.0767427087,
-0.132932663,
-0.4167051911,
-0.0587260388,
0.2930724919,
0.2494699806,
-0.1366453916,
-0.30391559,
0.1178889871,
0.1235293522,
-0.0146653019,
-0.0452341177,
-0.2453735173,
0.2158932239,
0.1646101475,
0.0025353599,
0.1477698833,
0.0169419814,
0.0003062184,
0.2684414387,
-0.039677199,
-0.2254716456,
-0.3118254542,
0.6033416986,
0.2232898474,
0.8363255262,
-0.2880607843,
-0.2029640079,
-0.2979713678,
-0.11180415,
-0.2330388427,
0.1009243056,
0.4950156212,
0.0988687202,
-0.04551965,
-0.4867722392,
-0.3448886871,
-0.0777857527,
-0.0465622693,
-0.2821798623,
-0.1423804462,
-0.2524194121,
0.1125408933,
0.1055419967,
-0.0284946524,
0.35754776,
-0.336345762,
-0.0157867335,
0.2345681489,
-0.3509430587,
-0.2011490315,
-0.1322091669,
0.5577652454,
0.12475954,
0.0052213185,
-0.3312214613,
-0.1804827899,
-0.1822399348,
0.0167687982,
0.1956835389,
0.3834738433,
-0.1756947488,
0.1844043136,
0.0359154977,
-0.3828607202,
-0.2349633425,
-0.1346767247,
0.1312392354,
-0.0012337214,
0.4762330353,
-0.4796628654,
-0.0986840948,
-0.3029204011,
0.170735389,
0.2444289774,
-0.096621953,
0.0273224898,
0.1169029027,
0.2148654461,
-0.2545850277,
0.2108534276,
-0.2304311395,
-0.1243431494,
-0.0487613045,
-0.1454731077,
-0.2492411137,
-0.0925649256,
-0.0672148913,
0.2685970962,
0.2856830955,
-0.2234886736,
0.3373496234,
0.027775988,
0.1063639969,
-0.141157046,
0.2592740953,
-0.3753346503,
0.3710653782,
0.4655984044,
-0.0162445158,
0.3749174774,
-0.1396909654,
0.1204947904,
-0.2708759308,
0.2553668916,
-0.5015743971,
-0.4222277105,
0.019115584,
0.1045882106,
-0.2029599994,
-0.0228900034,
-0.3181248009,
-0.0490738004,
0.4451743662,
0.2298894525,
0.233973816,
-0.2258068174,
-0.3481404483,
-0.2585401535,
-0.1718463749,
0.1132033318,
-0.3837776184,
0.2007804811,
0.0188008323,
0.2824502289,
0.0434071235,
0.4158513248,
-0.1315062642,
0.1567608863,
-0.0538132563,
-0.1209240332,
0.0304628313,
-0.1566626132,
-0.6271008849,
0.1202649027,
0.4168609083,
-0.027489027,
-0.1223236099,
0.4630418718,
0.0704056025,
-0.0158334002,
-0.109691754,
0.3284004033,
-0.1558928639,
-0.0901837051,
-0.2928343415,
-0.0386737064,
0.1061438695,
-0.0216424968,
0.1188712269,
-0.2305830568,
-0.1309050471,
0.1836872697,
0.0527518354,
0.1259610504,
0.0694234446,
0.3437758088,
-0.0101463888,
0.3748394549,
0.3232588172,
-0.0078654531,
-0.365346849,
0.257363379,
0.2419634461,
-0.6245028377,
0.1455274224,
-0.1339732707,
0.1272249073,
0.1450664252,
-0.0969082192,
-0.2118735611,
-0.0223335288,
0.029768955,
0.1151992679,
-0.210896045,
-0.0449688956,
0.5452651381,
-0.0635574982,
-0.110380061,
-0.418202579,
-0.1708040088,
0.0938118845,
0.0492022596,
-0.1359284222,
0.0650077462,
0.1010840386,
-0.0895124823,
-0.1746932715,
0.1081861779,
0.297341913,
-0.1439442486,
0.1077989191,
0.5784062743,
-0.0145125054,
0.1164005324,
0.4233711064,
-0.0106685152,
0.0905218571,
-0.1879726499,
-0.1697005033,
0.0567103922,
-0.1616249979,
0.1676455289,
-0.0029339031,
-0.0588440411,
0.0638453215,
0.1050605699,
0.187078014,
0.0860391632,
0.1927027106,
-0.0662448779,
0.3792729378,
0.4196599424,
-0.081911169,
0.1654994935,
0.4912528992,
-0.188942194,
0.0204285197,
0.1143536046,
-0.3488580585,
-0.4260420799,
0.0824614018,
0.3058717251,
0.4276179671,
0.091869235,
0.2786067128,
-0.0187048241,
0.1948913634,
-0.1964647472,
0.1110937074,
0.145748511,
-0.0842691883,
-0.2639130652,
0.1610331535,
-0.0088860849,
0.0190627202,
0.1868857294,
-0.153247118,
0.3150745928,
-0.0810951218,
0.4258529842,
-0.2198474854,
-0.0666334927,
-0.0421656519,
0.185401842,
-0.2399906963,
-0.2696066499,
-0.1647606194,
-0.091042541,
0.2942963243,
-0.1827508509,
-0.230092451,
0.4699299634,
0.0185605418,
0.0107688289,
0.1471360028,
-0.0653236657,
-0.3312748671,
0.064206183,
0.1377596557,
-0.2550902963,
0.2090717256,
0.0785146356,
0.0315915309,
-0.2303849012,
-0.158523947,
-0.19498761,
0.1075048223,
0.1837015748,
-0.2883615196,
0.0521756075,
-0.2645150721,
0.1547862589,
-0.2806054652,
-0.1142954454,
-0.0577976517,
0.1149196327,
-0.0509898253,
0.0269430727,
0.2000139952,
-0.0313187353,
-0.2764131427,
0.0005233336,
0.2373342067,
-0.0265396442,
0.0129479254,
0.2392877042,
-0.1557640433,
-0.0176598337,
-0.1436425745,
-0.0100039141,
-0.363374263,
-0.7905564308,
0.3151324391,
0.0385606065,
-0.1788749248,
0.2086086124,
0.1866609007,
0.0924994573,
0.1387996525,
-0.7085633278,
0.0724817142,
-0.3142879307,
0.2180023193,
0.0143604241,
0.0765084848,
0.1385976821,
-0.1808549762,
0.0806725398,
-0.114286378,
-0.2508398592,
-0.4035724401,
0.202921167,
0.3824835718,
-0.2886654735,
-0.0345724821,
0.2057443559,
0.4963883162,
0.557878077,
-0.0190252364,
-0.1098965555,
0.3746928275,
0.4645801485,
-0.3987116516,
-0.1185679957,
0.2061817646,
-0.1018319279,
-0.1222381219,
0.3536938429,
0.3558354676,
-0.0725569054,
0.2818091214,
-0.0484956689,
0.0352503955,
0.0698835254,
0.2234961092,
-0.2844492793,
0.1933543235,
0.1191815734,
-0.1655667126,
-0.1425039917,
-0.397328496,
-0.0735082999,
-0.0214168765,
0.0456137545,
0.2826975882,
-0.184295252,
-0.1279441416,
-0.0754016414,
0.1423415989,
-0.0231207386,
-0.0497247465,
0.0514619611,
0.2763727009,
0.3083381057,
0.3073396683,
0.3131420612,
-0.274462074,
-0.2138916701,
-0.1058373153,
-0.2411261201,
-0.1186881289,
-0.0318222903,
-0.0057501742,
0.301259011,
0.1512315273,
0.1316512525,
0.321107775,
-0.1440429389,
0.0087750554,
0.534434855,
-0.3888073564,
-0.2943490148,
-0.0840831175,
-0.2762682438,
0.2164512873,
-0.1252091229,
-0.1201286763,
-0.1507781595,
0.0234033149,
-0.0632728264,
-0.2404499799,
0.1136999503,
0.1901483685,
-0.1203188002,
0.1561103016,
0.2085816413,
0.0853591189,
0.1842174083,
0.1479958296,
0.1042302921,
0.3757860065,
-0.1913031936,
0.1126984656,
-0.0072585228,
0.0352628268,
0.277884841,
0.0563124865,
-0.1737894416,
-0.1832464933,
-0.2612355947,
0.0143590588,
-0.2691156566,
0.2237628251,
0.1496377289,
0.2776621878,
-0.2618526816,
-0.6982129812,
0.4616200924,
0.2764631808,
-0.0716559812,
0.2424005568,
-0.4183205664,
-0.0365162082,
-0.0543631129,
-0.0064192447,
0.6589257121,
-0.2236090899,
0.4306713045,
-0.0395674221,
0.0344166942,
0.4065585732,
-0.4473713338,
-0.028658079,
-0.0794860423,
-0.1845749468,
0.0040679458,
-0.1019873694,
-0.1771419793,
-0.0575258918,
-0.0451954044,
0.440168649,
-0.1805889904,
-0.0142156733,
0.0162403975,
0.1861698329,
0.043686986,
-0.1095274165,
-0.0421764068,
0.249251619,
-0.1284713,
-0.0425212234,
-0.0616239719,
0.1496698558,
0.0199737474,
0.1891243458,
-0.3140135109,
-0.2012456506,
-0.0288669057,
0.3245883286,
0.0098944511,
-0.1806677431,
-0.0580397472,
0.1913767159,
0.220396325,
0.2901022434,
-0.0961438641,
0.267059505,
-0.1876455843,
0.1555692255,
0.2435377836,
-0.0914695263,
0.1821190566,
-0.0270397607,
-0.0338976048,
0.0384036899,
0.0826622695,
-0.2128389776,
-0.1591346413,
0.3341324627,
0.0977139175,
-0.0641099811,
-0.2387067229,
0.0309947375,
0.1164923608,
0.0835460722,
0.1395623535,
0.3734088838,
-0.0597867779,
0.6573858261,
-0.2188907713,
-0.5179988742,
-0.0908970088,
-0.0430396497,
0.5688452125,
-0.246210441,
0.3715310395,
0.022857612,
-0.2190309167,
-0.0264743958,
0.2350379527,
0.0635286942,
0.1049834937,
0.1324854791,
-0.1333607584,
0.2987753451,
-0.1285156161,
-0.3920568228,
0.0370852165,
-0.3879739046,
-0.2860274017,
-0.1671658456,
-0.4105099142,
-0.0266917385,
-0.0878058746,
0.2242877334,
0.0568860918,
-0.2973289192,
0.1887331456,
0.4064843357,
-0.2433436513,
0.0819341391,
0.0297144838,
0.2258061618,
0.0675606951,
-0.0967761427,
0.0996916071,
-0.1272793859,
-0.1318951845,
-0.0946974531,
-0.1185578182,
-0.2398276925,
-0.0179096498,
0.1461031139,
0.17136316,
-0.0941339657,
-0.1098677516,
-0.3558127284,
0.1286488324,
-0.2562296987,
0.0847608298,
0.2919305265,
0.2346152067,
0.1277680099,
0.0235259756,
-0.2593018115,
0.1167763099,
0.3148724437,
0.0370092504,
0.2348183244,
0.1609543711,
0.1893200129,
0.2360896766,
-0.1818601489,
-0.2923297584,
0.0981743708,
-0.1392734796,
-0.1110361144,
0.1921978593,
-0.2242861837,
0.2773994803,
0.3868362308,
-0.1051516235,
0.2471596748,
-0.0514645651,
-0.1714697033,
0.1436868161,
0.1363580972,
0.1666207314,
-0.1629318297,
0.0704923794,
0.4474797249,
-0.2449587137,
0.1808711141,
0.1114569083,
-0.008500319,
-0.0219188835,
0.0780231431,
0.3410856724,
-0.3142292798,
0.1521827132,
0.0750651583,
0.1814655364,
-0.1232980788,
0.4441084266,
0.4823724031,
0.0159766041,
0.5705834031,
0.1776809543,
0.3709157407,
0.3553224802,
-0.2679767609,
-0.0616259202,
-0.3010145724,
-0.2353512347,
0.3864545226,
-0.1725270748,
0.0777342767,
-0.0796903968,
0.1531074345,
0.1485290676,
-0.3190406561,
-0.2061381042,
-0.0086432174,
-0.2841353118,
-0.1284307986,
0.4397258162,
0.060678795,
0.2145419717,
0.2614770532,
-0.0105379773,
-0.4243505895,
0.5057740808,
-0.0288448352,
-0.2109093368,
-0.2632436156,
0.2805338204,
0.1405213028,
-0.1363976747,
-0.100869067,
0.1854055524,
-0.2370547354,
-0.0349017531,
-0.1282426268,
0.0883427933,
0.2755080163,
0.1468667239,
0.0165943764,
0.3816765547,
0.0619968437,
-0.0660636798,
-0.1962118149,
0.179640606,
-0.2197112143,
-0.0932981297,
-0.0303407907,
0.1074645668,
0.0647099316,
0.2108063698,
-0.0653021187,
0.2227580547,
-0.3473086953,
-0.0111096781,
0.0606894977,
-0.0644293278,
-0.2514470816,
0.3058980107,
-0.4158520103,
-0.1779728383,
0.6756235957,
-0.1745560169,
0.0435055159,
-0.2043661028,
0.0827862769,
-0.2363874018,
0.5724009275,
0.5509406924,
0.184708491,
-0.2911016643,
0.0006311739,
-0.2649796009,
0.0914620981,
-0.474807322,
0.3963682652,
-0.0434778482,
0.4691887796,
-0.2120288163,
-0.0628108606,
-0.0016784242,
-0.0164434221,
-0.0396843776,
-0.1721838415,
-0.3049776554,
-0.0741594732,
0.0393010043,
0.1438495666,
0.0957856253,
-0.1642241478,
0.2386150807,
-0.3013194501,
-0.0327399075,
-0.1607343704,
0.0522633009,
0.163450703,
0.3378362358,
0.1687492281,
0.0903688893,
0.0849588513,
0.2190425694,
0.1733669788,
-0.3463304043,
-0.0603381582,
0.0893496573,
0.0750251412,
-0.0569980368,
-0.0385947786,
-0.5262832046,
0.1357942224,
0.1050977558,
0.155525133,
-0.1740638167,
-0.1851954311,
0.1313710511,
-0.3232075572,
-0.1827082187,
0.3909304738,
0.3479950428,
0.1791638732,
-0.1575753391,
-0.0387183465,
-0.191149056,
-0.0748234317,
0.3076616228,
-0.0438523665,
-0.3876530528,
-0.0505251512,
0.125873208,
0.4357411265,
-0.141032964,
-0.4110239744,
0.1245215759,
0.4666089714,
-0.0897404253,
-0.2220542878,
0.2676523924,
-0.1196449101,
0.1683702171,
-0.071762383,
0.5194827914,
-0.1256663948,
-0.0529368371,
0.2193594575,
-0.1838397384
]
|
https://github.com/huggingface/datasets/issues/1973 | Question: what gets stored in the datasets cache and why is it so huge? | Hi @ioana-blue, we have optimized Datasets' disk usage in the latest release v1.5.
Feel free to update your Datasets version
```shell
pip install -U datasets
```
and see if it better suits your needs. | I'm running several training jobs (around 10) with a relatively large dataset (3M samples). The datasets cache reached 178G and it seems really large. What is it stored in there and why is it so large? I don't think I noticed this problem before and seems to be related to the new version of the datasets library. Any insight? Thank you! | 34 | Question: what gets stored in the datasets cache and why is it so huge?
I'm running several training jobs (around 10) with a relatively large dataset (3M samples). The datasets cache reached 178G and it seems really large. What is it stored in there and why is it so large? I don't think I noticed this problem before and seems to be related to the new version of the datasets library. Any insight? Thank you!
Hi @ioana-blue, we have optimized Datasets' disk usage in the latest release v1.5.
Feel free to update your Datasets version
```shell
pip install -U datasets
```
and see if it better suits your needs. | [
-0.1255367398,
-0.0336778089,
-0.14823246,
0.5212610364,
0.1614961624,
0.2426844835,
-0.0925319791,
0.2584301233,
-0.0802799016,
-0.0226320326,
-0.0793154463,
-0.1993967891,
-0.0890248716,
-0.1937062144,
0.0600060374,
0.1441114396,
0.1028741077,
-0.0664708465,
-0.070582144,
-0.0968136191,
-0.1211708188,
0.0194597449,
0.0352943689,
-0.1679356247,
-0.5302936435,
-0.1817646772,
-0.1013381705,
-0.0436647758,
-0.165150106,
-0.1785152406,
0.1776832044,
-0.029039694,
0.317694068,
0.4055047333,
-0.0001109538,
-0.3099725544,
0.3714546561,
0.1150019988,
-0.4696369767,
0.3074621558,
-0.3643325567,
-0.3740324378,
-0.0264453273,
-0.1428352892,
0.3908942938,
0.0068974402,
-0.0497720987,
-0.421089083,
-0.0684007108,
0.1861254573,
0.2560692728,
-0.1310576946,
-0.3215966821,
0.1046228781,
0.119837068,
0.013891194,
-0.1468691677,
-0.2766183019,
0.2572325468,
0.3019107282,
-0.106885761,
0.2367847711,
0.061818596,
0.0476155579,
0.2798641324,
-0.0198586266,
-0.1303208023,
-0.1588062346,
0.6128360629,
0.2204000205,
0.9452200532,
-0.3251810074,
-0.1090440899,
-0.2270799726,
-0.1699434966,
-0.185639441,
0.2053112835,
0.3774013221,
0.0623727255,
-0.0036442082,
-0.4708915353,
-0.4204159975,
-0.1368878633,
-0.1069786698,
-0.1935634911,
-0.2392664701,
-0.2930276096,
0.0738700554,
0.2006106526,
-0.0358288065,
0.3408767581,
-0.2122139633,
-0.176235646,
0.1255209744,
-0.3456110954,
-0.2916094959,
-0.144486025,
0.4545298815,
0.1660124809,
0.0392153077,
-0.3247304559,
-0.1198162735,
-0.1323126853,
0.0058711222,
0.2860296667,
0.3430543542,
-0.0669391826,
0.1038032994,
-0.0429320522,
-0.3748683035,
-0.1334186047,
-0.1865021884,
0.1570679843,
0.0631489307,
0.444157958,
-0.5209649205,
-0.0817443803,
-0.3450356424,
0.1652761549,
0.1584218591,
0.0572483502,
-0.0358495377,
0.1435464919,
0.1911145747,
-0.1107612401,
0.2182201445,
-0.2996867299,
-0.214574486,
0.0177828725,
-0.1605704427,
-0.2281498015,
-0.1316340119,
-0.0949165374,
0.2396889925,
0.2744665742,
-0.16472736,
0.2371571809,
0.0634893104,
-0.0907147527,
-0.1422196925,
0.2349056154,
-0.4032540917,
0.3749281466,
0.4167664647,
-0.0743715614,
0.368103385,
-0.1970903724,
0.1527629048,
-0.227425918,
0.2449007779,
-0.4916739464,
-0.4740643203,
-0.1170704737,
0.1085805744,
-0.1619236022,
-0.0288122427,
-0.3449950218,
0.0922382548,
0.36340186,
0.1535674334,
0.160309732,
-0.0919574648,
-0.417909503,
-0.228961587,
-0.1571571827,
0.1740281433,
-0.4291955829,
0.1551631689,
-0.1355954558,
0.186601162,
0.0713288337,
0.3658940494,
-0.1201934889,
0.0764701962,
-0.0518554486,
-0.1532372087,
0.0406177714,
-0.105731979,
-0.6648840308,
0.1029136181,
0.3574775457,
-0.174062252,
-0.0367922634,
0.4561776221,
-0.0024712989,
-0.0370599926,
-0.2303043306,
0.2907509804,
-0.2054572701,
-0.1454720646,
-0.3278049827,
-0.0776618868,
0.0378574133,
-0.0154083557,
0.0659239069,
-0.2320690304,
-0.0371441543,
0.2797747254,
-0.0108112432,
0.1934455484,
0.1148359329,
0.3457118869,
0.0314271972,
0.354005903,
0.3015313745,
-0.0218940247,
-0.3611466885,
0.250936985,
0.235171482,
-0.7048802972,
0.1734371036,
-0.1321181804,
0.144807145,
0.1432245821,
-0.0207029451,
-0.1719020307,
-0.0045520165,
0.0870915279,
0.0700755268,
-0.1784891039,
-0.0182370823,
0.4595889747,
-0.0254145861,
-0.1279371828,
-0.3539278209,
-0.1449451298,
0.048782628,
0.0092003206,
-0.1537998021,
0.0313860998,
0.062304914,
-0.0768781081,
-0.1596941352,
0.1524796188,
0.2265720814,
-0.192876786,
0.2952666581,
0.5493301153,
-0.0663551018,
0.1707334071,
0.3852482438,
-0.0702986196,
0.1017342731,
-0.1299528927,
-0.2927915752,
-0.0375095829,
-0.1519739926,
0.1403202266,
-0.02493372,
-0.029297458,
0.0416709818,
0.0892700255,
0.3436175287,
0.0104555432,
0.1606139988,
-0.1470386237,
0.2927569151,
0.4069284499,
-0.024014052,
0.2519699335,
0.4782898426,
-0.1528939158,
0.0289913863,
0.1744275391,
-0.2387564629,
-0.5282303691,
0.1187323108,
0.378832221,
0.4037415683,
0.1002401263,
0.3153689206,
-0.1507233232,
0.2983992994,
-0.1419318616,
0.0769499019,
0.0800371841,
-0.0390973575,
-0.2749995291,
0.1334967613,
-0.0680699944,
-0.0156766623,
0.1987346411,
-0.0829685256,
0.3320787847,
-0.0365242139,
0.3400942981,
-0.2261351198,
-0.1101819128,
0.087478064,
0.3231964111,
-0.2059072405,
-0.2550527155,
-0.1116031632,
-0.0590512268,
0.2781716883,
-0.164449513,
-0.1198913902,
0.4901139736,
0.0762805045,
0.1016939208,
0.0700769648,
-0.0708680153,
-0.3164552748,
0.1030517817,
0.162566185,
-0.2699264884,
0.2397954464,
0.1029201746,
0.0852552876,
-0.3361774087,
-0.1510693133,
-0.1490522474,
0.1190941185,
0.2485795915,
-0.2534005642,
0.0126691032,
-0.3917894661,
0.1810094565,
-0.2936204374,
-0.1614061594,
-0.039520964,
0.1628921777,
-0.1019608676,
-0.0407593288,
0.1762524098,
0.0103806099,
-0.2491562665,
-0.0023533306,
0.2122996151,
-0.1259874105,
0.054906927,
0.2655704319,
-0.1126464158,
0.0555637069,
-0.1928710788,
-0.0497126468,
-0.3669956625,
-0.7784829736,
0.2513560355,
0.1395720094,
-0.1240603924,
0.1695471406,
0.2248828411,
-0.0192549191,
0.1598343551,
-0.7960988283,
0.0848228633,
-0.2673851848,
0.2629159689,
0.0260321684,
0.0380786471,
0.2360247374,
-0.1774864197,
0.0284533463,
-0.1528528929,
-0.2566245198,
-0.3062722385,
0.1367165595,
0.4193833172,
-0.2310950756,
-0.0271233562,
0.3263314962,
0.5199461579,
0.4282684326,
0.0144179538,
-0.0571796857,
0.3847502768,
0.5536596179,
-0.388767004,
-0.0796087086,
0.20065552,
-0.0727026984,
-0.0731621161,
0.3296692371,
0.3406891525,
-0.1232185736,
0.2847548127,
-0.0813003927,
0.0937905386,
0.1011781543,
0.1988085508,
-0.2678989768,
0.1599435508,
0.18611148,
-0.1608432233,
-0.0656140447,
-0.390984118,
-0.0692705363,
-0.0309387781,
0.0783747956,
0.2683599591,
-0.2041399628,
0.0029263517,
-0.0678284839,
0.1011183038,
-0.0287855025,
-0.006164846,
-0.0838761851,
0.3064675033,
0.2835106552,
0.2794950306,
0.3214493394,
-0.2633184791,
-0.0617114939,
-0.1646199077,
-0.1650079042,
-0.1018126905,
0.0064617721,
-0.0108330427,
0.2996634543,
0.083725065,
0.0862342715,
0.3078324199,
-0.1852035373,
0.0375437699,
0.4310307503,
-0.3830850124,
-0.3002844751,
0.0414094068,
-0.262549907,
0.2326454222,
-0.1294326484,
-0.1187387481,
-0.2153356969,
0.0714968666,
0.0059644305,
-0.2001734674,
0.1365023702,
0.1608419716,
-0.1547915488,
0.193230629,
0.2181782871,
0.1120680943,
0.1524928212,
0.1146081239,
0.0978650451,
0.2603717446,
-0.1108557284,
0.2093983889,
0.0343882255,
-0.0138657754,
0.1596276462,
0.0138399852,
-0.1994662583,
-0.1868090332,
-0.3193317652,
-0.0532790087,
-0.1618552804,
0.2261723429,
0.0621842407,
0.2645357549,
-0.2653850317,
-0.5795577168,
0.4742963016,
0.3399646878,
-0.1296412647,
0.2579105198,
-0.2862509191,
0.0161556304,
-0.0667421743,
0.0111748716,
0.5658610463,
-0.1947552711,
0.3254500628,
-0.0794113502,
-0.0616297126,
0.3023920059,
-0.4567987621,
-0.0652410239,
-0.0468616523,
-0.3045464754,
-0.0021179589,
-0.072098963,
-0.141113773,
-0.0199837703,
-0.0946817771,
0.3443317413,
-0.1653455049,
-0.0544737838,
0.1015559137,
0.1601573676,
0.0502448045,
-0.1256176382,
0.0404088907,
0.2785876989,
-0.1807654947,
-0.1113734916,
-0.0539933778,
0.1307121962,
0.0862979069,
0.2149005383,
-0.3181108534,
-0.2407792807,
0.0139594031,
0.355224967,
-0.0003475449,
-0.1252681017,
-0.0668670759,
0.1332378238,
0.2062504143,
0.2343955636,
-0.1449888498,
0.3111363351,
-0.1219341084,
0.1470762938,
0.2458628565,
-0.1360151768,
0.118489027,
-0.0817847773,
-0.0365060866,
-0.0434316173,
0.0705960095,
-0.2296568155,
-0.1445899904,
0.2853674889,
0.172163114,
-0.0119022764,
-0.2052930295,
0.035466291,
0.0315032266,
0.0794768333,
0.14196904,
0.3844062984,
-0.0609964468,
0.5599355102,
-0.2562154233,
-0.4541350901,
-0.0462833159,
0.0224628244,
0.5034425259,
-0.2178082764,
0.2553485334,
-0.0312377959,
-0.1767932177,
-0.0469375551,
0.2533655763,
0.060181886,
-0.0204420537,
0.1567869335,
-0.1809254438,
0.2217567563,
-0.141422689,
-0.4298367798,
0.0265336763,
-0.3845818639,
-0.2996764183,
-0.115665786,
-0.430551827,
-0.0555204861,
-0.233478263,
0.1969346553,
0.0284529682,
-0.4536218345,
0.1628563851,
0.4174414277,
-0.2616336644,
0.0988545716,
0.1829999685,
0.26799649,
0.0161524452,
-0.1439225674,
0.1268775612,
-0.1616187245,
-0.1091143116,
-0.1418680698,
-0.1457024217,
-0.2609520257,
0.0503478423,
0.1332999617,
0.1298935264,
-0.0771050155,
-0.1611855179,
-0.3070393801,
0.0476322919,
-0.2632961869,
0.0594223775,
0.3452679515,
0.2517390251,
0.0951138735,
0.1064111143,
-0.307300061,
0.1278261542,
0.3223632574,
0.0648431182,
0.2507063448,
0.0393300913,
0.2024415582,
0.1677876562,
-0.2629607618,
-0.3952639401,
0.1813608557,
-0.0667770579,
-0.115208365,
0.183922112,
-0.2410743386,
0.2093157619,
0.4576958716,
-0.0930895135,
0.1318605542,
-0.0612674356,
-0.2261503637,
0.0325866379,
0.187249288,
0.2583866119,
-0.1771099716,
0.0614770278,
0.4482593834,
-0.2375606745,
0.097470589,
0.1723155975,
-0.0793058276,
0.0926527232,
0.1511340886,
0.3712781072,
-0.348484695,
0.1774654239,
0.1149258092,
0.1882872134,
-0.1611398309,
0.4405623674,
0.465023905,
0.0315324515,
0.5609924197,
0.1833841205,
0.3716113269,
0.5011681318,
-0.191583097,
-0.1141738072,
-0.239333868,
-0.2015491724,
0.4385493696,
-0.1161763668,
0.0592843816,
-0.0714480132,
0.2083076984,
0.1838032752,
-0.3680932224,
-0.1831097007,
-0.1217204705,
-0.3449109495,
-0.1100356206,
0.4735719562,
0.0752971247,
0.1437613517,
0.2953137457,
-0.0625444204,
-0.3859573603,
0.4411940575,
-0.0149140181,
-0.1918068677,
-0.2876871824,
0.2943648398,
0.2762217522,
-0.1601945907,
-0.1219862625,
0.2891838253,
-0.2347111851,
-0.0451045111,
-0.0269342549,
0.133079797,
0.2197635472,
0.0857369825,
0.0260295924,
0.3150086403,
0.0979129672,
-0.1002512574,
-0.2087870389,
0.2268522531,
-0.2361117452,
-0.0908071697,
-0.0861887187,
0.1070383787,
0.0798825175,
0.2771607041,
-0.0013055328,
0.2785950601,
-0.2990991771,
-0.1270029843,
0.0565833487,
-0.0288750734,
-0.1554898769,
0.3027583659,
-0.3916992247,
-0.2383486331,
0.6524432898,
-0.2137705982,
0.0422888435,
-0.1606043875,
0.108450152,
-0.234999001,
0.44781515,
0.4689294398,
0.036987979,
-0.3087179363,
-0.0269653555,
-0.2480551153,
0.0657561421,
-0.3029731214,
0.4378801286,
-0.0127351517,
0.5008686185,
-0.239817515,
-0.0382047854,
-0.0074693095,
-0.0681988969,
-0.0351664461,
-0.1318752915,
-0.3061083257,
-0.0137973474,
0.1377976686,
0.1543893218,
0.0681297854,
-0.2035731077,
0.2141357809,
-0.2355101854,
-0.02890517,
-0.1541023552,
-0.0110403858,
0.1529421061,
0.4067370892,
0.0864680558,
0.1257699281,
-0.0493975542,
0.1526640356,
0.1546428949,
-0.3914330602,
-0.0762769356,
0.0519486815,
-0.0218606964,
0.0463793688,
0.0035660432,
-0.5463690758,
0.2738309801,
0.1344442666,
0.1515660733,
-0.1615918726,
0.0087533239,
0.1073764861,
-0.3834522665,
-0.2046898305,
0.3414939642,
0.312323451,
0.1437020153,
-0.0722698793,
-0.0394231826,
-0.3186280429,
-0.0789775625,
0.3032260537,
0.0869202018,
-0.4065344334,
-0.0037892973,
0.1015671864,
0.5180826187,
-0.1703475565,
-0.3908232152,
0.1476502717,
0.4772285819,
-0.1026959568,
-0.1970787048,
0.2607144415,
-0.1244608015,
0.200963974,
-0.0636364371,
0.5886347294,
-0.0591426082,
-0.0374849103,
0.2227339149,
-0.1312393844
]
|
https://github.com/huggingface/datasets/issues/1965 | Can we parallelized the add_faiss_index process over dataset shards ? | Hi !
As far as I know not all faiss indexes can be computed in parallel and then merged.
For example [here](https://github.com/facebookresearch/faiss/wiki/Special-operations-on-indexes#splitting-and-merging-indexes) is is mentioned that only IndexIVF indexes can be merged.
Moreover faiss already works using multithreading to parallelize the workload over your different CPU cores. You can find more info [here](https://github.com/facebookresearch/faiss/wiki/Threads-and-asynchronous-calls#internal-threading)
So I feel like the gains we would get by implementing a parallel `add_faiss_index` would not be that important, but let me know what you think.
| I am thinking of making the **add_faiss_index** process faster. What if we run the add_faiss_index process on separate dataset shards and then combine them before (dataset.concatenate) saving the faiss.index file ?
I feel theoretically this will reduce the accuracy of retrieval since it affects the indexing process.
@lhoestq
| 79 | Can we parallelized the add_faiss_index process over dataset shards ?
I am thinking of making the **add_faiss_index** process faster. What if we run the add_faiss_index process on separate dataset shards and then combine them before (dataset.concatenate) saving the faiss.index file ?
I feel theoretically this will reduce the accuracy of retrieval since it affects the indexing process.
@lhoestq
Hi !
As far as I know not all faiss indexes can be computed in parallel and then merged.
For example [here](https://github.com/facebookresearch/faiss/wiki/Special-operations-on-indexes#splitting-and-merging-indexes) is is mentioned that only IndexIVF indexes can be merged.
Moreover faiss already works using multithreading to parallelize the workload over your different CPU cores. You can find more info [here](https://github.com/facebookresearch/faiss/wiki/Threads-and-asynchronous-calls#internal-threading)
So I feel like the gains we would get by implementing a parallel `add_faiss_index` would not be that important, but let me know what you think.
| [
-0.2789410353,
-0.0627319962,
-0.1573459953,
0.1106121838,
-0.3771445751,
0.2478515506,
0.2097887248,
0.1062705591,
0.1371022165,
0.1719248295,
-0.1766027659,
-0.1353846043,
0.3414870501,
0.1139963791,
-0.3853701353,
0.2495386302,
0.351341486,
-0.103391543,
0.2524389029,
0.1125721261,
-0.3173969984,
0.0103293927,
0.0291405004,
-0.267421633,
-0.1780145764,
0.3508559763,
-0.2582989037,
0.0532394946,
-0.0132863186,
-0.2483889312,
-0.2480454594,
0.3926288188,
0.0679371208,
0.3004918993,
-0.0001269445,
-0.0502258576,
0.1069198847,
0.069117859,
0.153345257,
0.5967575312,
-0.2711211741,
0.0120972507,
-0.0762950033,
-0.1729464829,
-0.0013869638,
-0.3030719459,
-0.0339292809,
-0.2620635629,
-0.2039727718,
-0.1719792783,
-0.0102491137,
-0.1237886176,
0.0848587081,
0.0481444225,
-0.0428055711,
-0.2038856298,
-0.1553708315,
-0.1455569267,
0.0888658613,
0.2237186581,
0.2321638316,
0.1709750295,
0.0189925041,
-0.1704599559,
0.0877618939,
-0.0448629782,
0.4525159001,
-0.0526514053,
0.0105196666,
-0.1225786284,
0.1086313576,
0.0454837345,
-0.4651237428,
-0.2125894874,
0.0684299842,
0.0824427903,
-0.227445364,
-0.1753317565,
0.039013654,
0.0092716925,
0.0714065731,
-0.4730631113,
0.0494286381,
0.1115153134,
0.3911834657,
0.0608309247,
0.2611272335,
0.1117505431,
0.4002322257,
-0.1047058627,
0.0262905546,
-0.1128799245,
-0.0963884145,
0.1314447224,
-0.6142690778,
0.023287598,
-0.0899241865,
-0.2996665537,
0.020942213,
-0.1793350726,
-0.3022100031,
0.2885175049,
0.0202775598,
0.1198160499,
0.0016665775,
-0.1536526829,
0.2066200972,
-0.250123173,
-0.0125040952,
-0.4881712794,
-0.1144801527,
0.137448296,
0.1478853971,
-0.1201161146,
-0.6300250888,
-0.1439968646,
-0.3549612164,
-0.1606157124,
-0.0620710775,
-0.1819742173,
0.0238425471,
-0.0995018855,
0.1697279811,
0.1614186615,
0.3377498686,
-0.0016710648,
-0.1338704079,
0.0112253781,
-0.0672989786,
-0.0540433452,
-0.0022989975,
-0.2795438468,
0.243451491,
0.5302842855,
-0.0042610914,
-0.2697485983,
-0.4165174365,
-0.027876541,
-0.0764701143,
0.1563665569,
0.0336072147,
-0.1561208069,
0.0336155966,
0.0777925029,
-0.0235533565,
0.0020425911,
-0.0585455298,
0.1687942445,
-0.2429048419,
0.1656705141,
-0.2159647048,
-0.3029151559,
0.0556754619,
0.005408824,
-0.0320963487,
-0.1371089071,
-0.1657651663,
0.5699168444,
0.1761475205,
-0.043269787,
-0.0384707972,
0.1838903576,
-0.3282157779,
-0.1131455749,
0.1609256268,
0.1564362794,
-0.2961852849,
-0.2146447748,
-0.1436221153,
-0.1857853681,
0.0067236843,
0.570731163,
-0.0211947039,
0.0817073509,
-0.2875503898,
0.5438025594,
0.2934735715,
-0.2815659046,
0.0505189821,
0.0885653198,
-0.0611449629,
-0.1653108895,
0.0520845167,
0.1922507882,
0.1179278716,
-0.0568648875,
0.2276800126,
0.2944650352,
-0.0839013606,
-0.1194113418,
-0.2615700066,
-0.3065001965,
0.4936807454,
0.24300915,
-0.1071244925,
0.0761089399,
0.0439902358,
-0.7627868652,
0.286003679,
-0.1323406696,
0.1944719255,
0.0383248925,
0.2891153395,
0.4427098334,
0.4458392262,
-0.1140968278,
0.0472650081,
0.2294327915,
-0.1534681916,
-0.0019356721,
0.3032389879,
-0.3450815678,
0.4740670025,
-0.0507175475,
0.030564107,
0.3955186903,
-0.0676674917,
-0.2117540538,
0.1425753236,
-0.3888502717,
-0.3314332068,
0.465803355,
-0.3955698609,
-0.1659500748,
-0.0724600255,
0.2263810486,
-0.031209819,
-0.0337085202,
-0.3186899424,
0.095929198,
0.1591543704,
-0.2405869812,
-0.1059324518,
0.3029583693,
0.0247300509,
0.0376864076,
0.9103010893,
0.2225019336,
-0.0706848651,
0.2421092391,
0.058199469,
-0.259522289,
-0.1585792154,
-0.1893134564,
0.3330982327,
0.0545158759,
0.0887112468,
0.5726434588,
0.0026581266,
-0.1720756888,
0.1380842775,
0.1619299352,
0.0660992861,
-0.024327755,
-0.0152290007,
-0.126603201,
-0.113096416,
0.0886518285,
-0.0277292244,
0.5910166502,
-0.2684079707,
-0.082285732,
-0.1726531088,
-0.0034398001,
-0.0326989293,
0.0887019709,
-0.0420207493,
-0.194868505,
0.312199086,
0.1168775484,
0.0520308018,
-0.158430472,
0.3804953396,
-0.1136475205,
-0.0397200733,
0.363324374,
0.2026495934,
0.0750138462,
0.1684561968,
-0.0580854937,
-0.0571015924,
-0.1315050125,
0.0688442811,
0.0319430232,
-0.0110609047,
-0.0850688517,
0.1229410246,
0.0800906271,
-0.1491920203,
-0.2043135911,
-0.3969108462,
-0.196898371,
0.2237751931,
0.0126259411,
-0.2535371184,
-0.0580513403,
-0.0775194764,
0.5329556465,
-0.0946547613,
-0.2684037685,
-0.3124781847,
-0.0170468967,
0.1478614509,
-0.1259401739,
0.2970302701,
0.1887923181,
-0.0532314964,
0.1188509837,
-0.0868264437,
-0.3709446192,
-0.0934977829,
-0.1448414624,
-0.0173403602,
-0.1443889141,
-0.2989168465,
-0.2838768363,
-0.1429503113,
-0.164475739,
0.1537100375,
-0.0450637452,
-0.096455127,
-0.2686012387,
0.0291596223,
0.0496854298,
0.1025892496,
0.0748391375,
-0.3937213123,
-0.2396275699,
0.3449152708,
-0.0618614666,
0.0971541777,
-0.353382796,
-0.288266927,
-0.1973518878,
-0.2623564601,
-0.2343727648,
-0.3656176925,
0.0202944912,
0.1619837731,
-0.1117467508,
-0.1317773461,
0.0018891779,
0.0815019831,
-0.2325872928,
0.3353892863,
0.0072119348,
0.0734023526,
-0.0066111949,
0.1284050196,
-0.2605769932,
0.1623672694,
0.1447143853,
-0.1115816608,
-0.0068714293,
0.0925004408,
0.1554602981,
0.2978829443,
0.1614470333,
0.1604870856,
-0.1416186392,
-0.0257231854,
-0.1567706913,
0.7340025902,
0.257725805,
-0.0254652388,
0.1525014788,
0.117744863,
0.0364690274,
0.0644163713,
-0.1403582245,
0.2962309122,
0.0880494118,
-0.1183746904,
0.0249203164,
0.0486863181,
-0.0981475115,
0.0440148935,
-0.1993703395,
-0.1111700907,
-0.1187807843,
-0.0568944998,
0.2207624316,
0.5126083493,
0.0025171821,
-0.0549470857,
-0.2808343172,
0.0094223451,
0.1832816601,
0.3620159924,
0.4992682636,
-0.1927377135,
0.1517201662,
-0.2025242299,
-0.4655701816,
0.4637282789,
0.0087807057,
0.2816376388,
0.0165973883,
-0.044791095,
0.2494058311,
-0.1914136708,
0.890162766,
0.0034869928,
-0.4772206247,
0.113479279,
-0.0452142768,
-0.3266534507,
-0.2326374203,
0.3000527322,
0.2172365636,
0.4511133432,
0.2308521569,
-0.2460670024,
-0.3018378317,
0.1756523997,
0.0751906559,
-0.2882427871,
-0.5182566643,
-0.1469022632,
0.0661564395,
-0.0823279843,
0.1156660095,
-0.2963602543,
0.1139921471,
0.0361340977,
0.0092623113,
-0.1929905564,
0.0298596397,
-0.0371089689,
0.0602861941,
0.0510644205,
0.1120234132,
0.4447425008,
0.3219394982,
-0.2890277207,
0.2662294805,
0.0822310895,
0.328691572,
0.0109015508,
-0.0902362838,
-0.0044669667,
0.2150544822,
0.0719430149,
0.0639623329,
0.2853555083,
-0.121838361,
0.2022847235,
-0.2137942165,
0.1298510283,
0.1342013925,
0.2930412591,
-0.1915014982,
-0.173327893,
0.4962489605,
0.044203002,
-0.1058535203,
-0.0767580047,
0.4734344482,
-0.0099866241,
0.714695096,
0.0455129072,
0.7037382722,
-0.1248850897,
-0.1526595801,
-0.2615526915,
0.1158450022,
0.0420728549,
-0.6474254131,
0.3106972575,
-0.1907193065,
-0.2052680403,
0.1221540123,
-0.1048922464,
0.1149577498,
0.3697926998,
0.279747963,
0.1120953485,
0.2166122347,
-0.2035366297,
-0.2071073502,
0.3400912583,
0.1826966405,
-0.2283104956,
0.2803767025,
0.0391107574,
0.0797609538,
-0.030665718,
0.0226754751,
0.061544802,
-0.1580806375,
0.3526023924,
-0.1197993532,
-0.0483022965,
0.326513648,
0.2986388505,
-0.2302101701,
-0.0455087833,
0.1209438145,
-0.5679503679,
0.2275298238,
-0.2574242353,
-0.1487647295,
0.1044513062,
0.1428870708,
-0.1538692564,
-0.0604205392,
-0.5270252228,
-0.0879808813,
0.0198986065,
-0.304712832,
-0.1901981831,
0.2688497305,
-0.8522257805,
-0.388807714,
0.0930699036,
-0.0728900656,
0.0655891448,
-0.126383394,
0.5215660334,
-0.1912917942,
0.0358546153,
-0.0476522185,
0.2592446506,
-0.4425131679,
0.7070085406,
-0.3910200894,
-0.0085429642,
-0.0221073739,
0.243318364,
0.2357961684,
-0.3659253418,
0.0845106319,
-0.0970673263,
-0.1915615052,
0.039833948,
-0.5570605397,
0.0905362591,
0.4957047701,
-0.0826074407,
0.0287383795,
-0.1235793009,
0.0528360941,
-0.1890912056,
-0.1416684836,
-0.0433611311,
-0.6022045016,
-0.1440007091,
-0.2501830161,
0.0947241858,
0.2008907497,
0.3670534492,
0.136548236,
0.1150725707,
-0.3318487406,
0.1516890675,
-0.1088536829,
-0.0053227246,
0.1221120059,
0.0959042758,
-0.0204844903,
-0.0927879885,
0.1320213377,
-0.0813643634,
-0.090085119,
0.1627067327,
0.1668040305,
-0.0654662549,
0.2371115088,
0.2261744291,
0.3129312992,
0.1566812396,
-0.1954315007,
-0.2620933354,
-0.1907633394,
-0.2348841578,
0.044474896,
0.0386032909,
-0.2214901298,
-0.0736929476,
0.3863518834,
-0.2685997486,
0.1082158461,
0.5082781911,
0.4393988848,
0.4513733983,
-0.0027624231,
0.5174338222,
-0.2549320757,
0.0965868384,
0.1337030977,
0.049998939,
0.2449608892,
0.0504373312,
0.0262856018,
-0.0082347794,
-0.2804999352,
-0.0818448141,
0.489674896,
0.6631746888,
0.2678462267,
-0.2227075249,
-0.0256316215,
0.0075579919,
-0.048143208,
-0.0765162483,
0.839158237,
-0.1111107916,
0.1397630572,
0.1743852496,
0.3427317441,
0.3477386534,
-0.2052408159,
0.0624892302,
0.0630098879,
0.3767136037,
-0.14992401,
-0.6269668341,
0.231636852,
0.0486396179,
0.2675713599,
-0.15359658,
0.124084495,
0.7165518999,
-0.063914448,
0.4785978794,
0.0993160084,
-0.0497427769,
0.1411429495,
-0.1296243966,
0.4695599079,
0.1944604963,
0.3647834361,
0.169102326,
0.1554944813,
0.2924838662,
-0.0987270772,
-0.5738972425,
-0.1505663544,
0.4674295783,
-0.0120794661,
-0.045410879,
0.4208973348,
0.0418875143,
-0.0121287601,
0.0280937012,
0.1323242635,
-0.1338594556,
0.194598496,
-0.0022144029,
0.1079650894,
0.2100121826,
-0.0201817136,
0.4173365831,
0.4710711837,
-0.0277035628,
-0.2999331951,
-0.0784053206,
-0.0858178139,
0.1237094924,
0.1651204526,
-0.0054089166,
0.1638240069,
-0.010343316,
0.3054113388,
-0.0628253892,
0.1377595961,
0.0035015089,
-0.0520886183,
-0.310949862,
-0.187294662,
0.2082957178,
-0.0313970298,
-0.0078560794,
-0.572486639,
-0.1981352121,
0.4705941379,
-0.1560833752,
-0.0974817127,
0.097962603,
-0.0830198377,
-0.0531943813,
-0.080322884,
-0.0383727811,
-0.2392768413,
0.0729021132,
-0.4864428639,
-0.2075454146,
0.1342897266,
-0.0296102371,
-0.1673310399,
0.3975626528,
0.2318828404,
0.5182496309,
-0.1991003752,
-0.1807958633,
-0.2487401366,
0.1420688182,
-0.142135039,
0.3399437368,
0.0479400195,
0.5490871668,
0.0789261386,
-0.0603775755,
0.6871289015,
0.1367734671,
0.1093956009,
0.463611275,
-0.1878230274,
0.3570117652,
-0.3443449438,
-0.284706533,
0.13399975,
-0.0340750813,
0.4974753559,
0.2011551112,
-0.2478419244,
0.2226830125,
-0.2510330677,
0.3260992169,
-0.4909693599,
0.5764991641,
0.0635077581,
0.1297812015,
-0.0798648968,
0.0456422605,
-0.1233183891,
-0.2051601112,
-0.3342984617,
0.0662150159,
-0.3860466778,
-0.2056216151,
-0.2810481787,
-0.1591936797,
0.0470139608,
0.2368545532,
-0.1396908611,
-0.1990496367,
-0.060356997,
0.0884798616,
-0.1346511245,
0.2249331176,
-0.0805845931,
0.1380124539,
0.1908538193,
0.2626540065,
-0.2852629721,
0.280865103,
0.2794202864,
-0.181610465,
-0.0213586949,
-0.4958340228,
0.1355201155,
0.405075103,
0.1090614423,
-0.524743557,
-0.529317081,
0.1268746853,
0.3336170316,
0.2443784773,
0.1576972008,
-0.0107486397,
-0.3092317879,
-0.2780921459,
-0.3314500153,
0.0037304198,
0.197345823,
0.0342445783,
-0.033960633
]
|
https://github.com/huggingface/datasets/issues/1965 | Can we parallelized the add_faiss_index process over dataset shards ? | Actually, you are right. I also had the same idea. I am trying this in the context of end-ton-end retrieval training in RAG. So far I have parallelized the embedding re-computation within the training loop by using datasets shards.
Then I was thinking of can I calculate the indexes for each shard and combined them with **concatenate** before I save. | I am thinking of making the **add_faiss_index** process faster. What if we run the add_faiss_index process on separate dataset shards and then combine them before (dataset.concatenate) saving the faiss.index file ?
I feel theoretically this will reduce the accuracy of retrieval since it affects the indexing process.
@lhoestq
| 60 | Can we parallelized the add_faiss_index process over dataset shards ?
I am thinking of making the **add_faiss_index** process faster. What if we run the add_faiss_index process on separate dataset shards and then combine them before (dataset.concatenate) saving the faiss.index file ?
I feel theoretically this will reduce the accuracy of retrieval since it affects the indexing process.
@lhoestq
Actually, you are right. I also had the same idea. I am trying this in the context of end-ton-end retrieval training in RAG. So far I have parallelized the embedding re-computation within the training loop by using datasets shards.
Then I was thinking of can I calculate the indexes for each shard and combined them with **concatenate** before I save. | [
-0.2849660218,
-0.1696968824,
-0.0912609622,
0.1680027097,
-0.3235115707,
0.3758882582,
0.3347997665,
0.06972038,
-0.035886772,
0.1546952128,
-0.0876064822,
0.0618727617,
0.3824172914,
-0.020076016,
-0.3032029569,
0.1434957534,
0.2809818983,
-0.0584645979,
0.2126168758,
0.039479278,
-0.3338528275,
-0.1382641494,
-0.0715477467,
-0.2807030678,
-0.2238434106,
0.1862553209,
-0.3562580943,
0.1115743667,
-0.00082417,
-0.3895471096,
-0.1900934726,
0.3138125539,
0.2559102178,
0.0299703162,
-0.0001277621,
-0.0997196287,
0.1779815406,
0.0183814,
0.1161233038,
0.6891211271,
-0.2972021997,
-0.0267104097,
-0.0404045284,
-0.2445940226,
0.0822641775,
-0.2505578697,
0.0011065376,
-0.2517292798,
-0.0702407137,
-0.2690675259,
-0.0466796979,
-0.0607915372,
0.0575929321,
0.0574583858,
0.0406845771,
-0.3079019785,
-0.1213594154,
0.1398369968,
0.0839521885,
0.2245055735,
0.1343076527,
0.0507927686,
-0.0477381274,
-0.1600026488,
0.0726075843,
0.1129784212,
0.2452799082,
0.1347257197,
-0.05123882,
-0.1212151051,
0.2552975416,
-0.0992786735,
-0.534969151,
-0.158233121,
0.2663681507,
-0.1595624983,
-0.1438804418,
-0.1659994423,
0.1047990769,
0.0622216947,
0.0662735626,
-0.5065564513,
-0.0104388213,
0.0325584933,
0.4056908786,
0.0920156687,
0.2955590487,
0.1298531741,
0.3458766341,
0.0445572585,
0.0997551829,
-0.202911377,
-0.1997182965,
0.1131247729,
-0.6591155529,
-0.0331773758,
-0.2063945085,
-0.2228664458,
-0.1241204441,
-0.0351019427,
-0.1060765907,
0.1871546209,
0.2389023751,
0.1609720886,
-0.0058838199,
-0.0895851403,
0.0343845412,
-0.1298582405,
-0.1368227154,
-0.4281221628,
-0.1075875908,
0.0488345623,
0.0350250527,
-0.1958868951,
-0.5263503194,
-0.0633084401,
-0.4865526855,
-0.071992971,
-0.1026424617,
-0.1734398752,
-0.1677079648,
-0.1291920096,
0.1297934055,
0.0206171218,
0.2822859585,
-0.1592239141,
-0.1701837182,
0.0673737228,
-0.055986952,
0.0045552785,
-0.0279972702,
-0.2161834538,
0.0174259376,
0.5126779675,
-0.0436443761,
-0.2863789201,
-0.3827866316,
0.0505873822,
-0.0481580384,
0.1748679727,
0.0664138198,
-0.2154342979,
0.120925121,
0.0772428438,
-0.1290805489,
-0.1677448303,
-0.0365749262,
0.2454082817,
-0.1966934353,
0.2098248154,
-0.2311401367,
-0.2099298388,
0.164612636,
-0.0386468694,
-0.0348174497,
-0.1441638619,
-0.0446498953,
0.335272342,
0.3445597291,
-0.0064370343,
0.0062950822,
0.1591218263,
-0.2874040902,
-0.1396418661,
0.2494934201,
0.2338723391,
-0.401492089,
-0.1169340909,
-0.0487823151,
0.0194944888,
0.1672156453,
0.5547193289,
-0.1314318329,
0.1645197868,
-0.3813925683,
0.5477819443,
0.3399353027,
-0.282605648,
-0.0182862803,
0.0328421891,
-0.1953349262,
-0.096134223,
0.2637236118,
0.1584375501,
0.4460000396,
0.0293703564,
0.2704949975,
0.2283855081,
-0.066930376,
-0.3063271046,
-0.3454910815,
-0.2478903532,
0.2212375402,
0.141330108,
-0.0717566013,
0.1985466927,
0.0511375442,
-0.8309344649,
0.3635241389,
-0.084066622,
0.2159192562,
-0.0107424576,
0.2092398405,
0.3811726272,
0.3770608306,
-0.0781182647,
0.0771493316,
0.159985736,
-0.0280743986,
-0.0409755521,
0.2488019168,
-0.3538299501,
0.483163327,
-0.075630717,
0.0241908059,
0.4689190984,
-0.1199093163,
-0.2062517107,
0.0621982925,
-0.3314240575,
-0.2941705287,
0.5498024225,
-0.4779764414,
-0.0968440846,
-0.1887804717,
0.2802513242,
0.0779901743,
-0.1488748938,
-0.2816526592,
0.0475952812,
0.0733577535,
-0.2442056239,
-0.0596470572,
0.2386411875,
-0.0809267387,
0.1019928604,
0.8778347969,
0.1095692813,
-0.1541575044,
0.071127452,
0.1545335054,
-0.3248100877,
-0.0852530971,
-0.2058341056,
0.3224864006,
0.2268131375,
0.02490367,
0.360970825,
0.0308595207,
-0.0908335149,
0.0195495933,
0.0234933682,
0.0144608486,
0.0800581798,
-0.10248404,
0.0013244258,
-0.0705541745,
0.045099318,
-0.112069428,
0.3265724778,
-0.1385031343,
-0.1785279363,
-0.2923912406,
-0.05336551,
-0.0340082832,
0.1097715423,
-0.0718639269,
-0.1530021578,
0.3807483912,
0.1627700478,
0.1704764813,
-0.1295462698,
0.2641076744,
-0.1942447275,
0.024381347,
0.2502624393,
0.0645521134,
0.2118135691,
0.0696620271,
-0.0877098888,
-0.0326041766,
-0.0260462128,
0.092872642,
0.0639978647,
-0.0158803817,
-0.0416264236,
0.0864129141,
0.3875239491,
-0.3104659617,
-0.1104386374,
-0.2621285915,
-0.168506667,
0.1309843361,
0.0351333618,
-0.1759461313,
-0.0140685113,
-0.0472525842,
0.5214403868,
-0.1426886767,
-0.3105710149,
-0.1479192674,
-0.1127291322,
0.119670108,
-0.1296889037,
0.2577826381,
0.1593423486,
0.1317598373,
0.1190095991,
-0.04782423,
-0.2820172608,
0.0005476919,
-0.1702172458,
0.0225035157,
-0.2009871602,
-0.302567929,
-0.2304279059,
-0.3104276955,
-0.121389173,
0.0695400834,
0.1559572369,
-0.0151105002,
-0.2252913117,
0.113005206,
0.0651465431,
0.1385293305,
-0.137159586,
-0.3187464178,
-0.2540184259,
0.3161887228,
-0.1976725906,
-0.0086671524,
-0.4010066986,
-0.1311910599,
-0.1447113007,
-0.1944412887,
-0.2484068573,
-0.3096929193,
-0.172685951,
0.152539745,
-0.026311392,
-0.0767532736,
-0.0058420794,
-0.0332636125,
-0.1281506866,
0.6561332345,
-0.0107418159,
0.2173006684,
0.0476657972,
0.0933472589,
-0.2506474257,
0.2637115419,
0.2129896283,
-0.0281588454,
0.0079064537,
0.1556271613,
0.294721067,
0.369546622,
0.0974453464,
0.0661056489,
0.0408574529,
0.0288636684,
-0.193277657,
0.9015146494,
0.2490783483,
-0.1850934625,
0.1608605683,
0.259434551,
0.1495264024,
0.007931333,
-0.1160739958,
0.2399919182,
0.1435942203,
-0.1371480227,
0.000988926,
-0.0364589654,
-0.0945904031,
0.1096183732,
-0.2012954056,
0.0115333078,
-0.1715346426,
-0.0033564677,
0.0544864722,
0.5516694188,
0.0550485477,
0.0214145314,
-0.2025680095,
0.0437315963,
0.1283032596,
0.3945730031,
0.5861865878,
-0.2756179571,
-0.0562681332,
-0.2580191195,
-0.301384896,
0.4053606987,
0.0661223233,
0.3546912074,
0.1334125847,
-0.1077653766,
0.3340644538,
-0.115537487,
0.897454977,
-0.131309256,
-0.5274401903,
0.1343754083,
0.0533273891,
-0.1458679587,
-0.2606737316,
0.3020032644,
0.1860319972,
0.3533157706,
0.1942867488,
-0.2180703878,
-0.2082675397,
0.3316383064,
0.1343442351,
-0.2656055093,
-0.652995944,
-0.0941176862,
0.1721440703,
-0.0474794805,
0.2200228721,
-0.3541428149,
0.0357386097,
0.0266459808,
-0.0205886755,
-0.3798433542,
-0.0183667373,
0.0105701825,
-0.0418419838,
0.0875429213,
0.0219411347,
0.5158407688,
0.3603675961,
-0.0402312502,
0.426327467,
0.0963509157,
0.0793372393,
0.0130315041,
-0.1868697405,
-0.1968353838,
0.3067003191,
0.0957240239,
0.0654581785,
0.3111416996,
-0.2788873017,
0.0630098656,
-0.3485803008,
0.0565939173,
0.1023264155,
0.4389439821,
-0.1225832701,
-0.1930825859,
0.4389563799,
0.0504129715,
-0.1514481008,
-0.0545304939,
0.3455344737,
-0.1894800067,
0.6812266707,
0.0664829835,
0.8806685209,
-0.1594640762,
0.0483081229,
-0.2123779207,
0.0975700691,
-0.0178217925,
-0.6159814,
0.4225198925,
-0.2377067208,
-0.1771935076,
0.104971461,
-0.2092420757,
0.2017689645,
0.4122807086,
0.1896035522,
0.1242856234,
-0.0764311552,
-0.0992065668,
-0.1765963584,
0.2509701252,
0.2230388373,
-0.2394241989,
0.344689548,
-0.0590550974,
0.2382805794,
-0.2323366404,
0.0191421118,
0.0264443457,
-0.1703361869,
0.3796363175,
-0.1086361259,
-0.0167272408,
0.448112011,
0.1966138631,
-0.173183322,
-0.2193168998,
0.2574955523,
-0.3695750237,
0.3011196554,
-0.2077327818,
-0.0317818634,
-0.0070876237,
-0.0048744259,
-0.2725215256,
-0.1024237648,
-0.3807837665,
-0.0331251658,
0.1110460311,
-0.3711656332,
-0.1661392599,
0.263186723,
-0.7294943333,
-0.4386254549,
0.1284068525,
-0.0531709753,
-0.1192604527,
-0.1365407109,
0.4132650197,
-0.1144071594,
0.0019171992,
-0.0689255819,
0.2600788474,
-0.4817505777,
0.8244268894,
-0.3634016812,
0.0254215319,
0.0193001181,
0.2881210446,
0.312102139,
-0.2579219639,
0.2277694196,
-0.1517079771,
-0.234892875,
-0.0000380295,
-0.5809665918,
0.1541990936,
0.4484094977,
-0.0926694125,
0.0182481371,
-0.0281315502,
-0.0096790884,
-0.4759544134,
-0.0965824798,
0.0260912385,
-0.5716091394,
-0.127910912,
-0.3554805219,
0.101309441,
0.2063253969,
0.4299416244,
0.0584560931,
0.1112203002,
-0.3822533488,
0.1869218498,
-0.1091271564,
0.0534865409,
0.1014931127,
0.2128514946,
0.1496150047,
-0.0973925889,
0.1051632762,
-0.0466012321,
-0.0639348999,
0.1806937158,
0.098582007,
-0.0267343447,
0.287789464,
0.2327820212,
0.1426941007,
0.1709013581,
-0.0853706822,
-0.3187398911,
-0.2249474376,
-0.2446720749,
-0.0246263333,
-0.0533239692,
-0.1381592453,
-0.0823492259,
0.4531772733,
-0.0884271264,
0.0370722376,
0.452721715,
0.3983289897,
0.3977433145,
-0.0550011024,
0.4254107475,
-0.2170280516,
0.0043881186,
0.0188510865,
0.1060652062,
0.3288203478,
0.0073820404,
0.1000650898,
-0.0175164491,
-0.3024100363,
-0.2244686931,
0.5101445913,
0.6108432412,
0.3429230154,
-0.4377303421,
0.1114996895,
-0.0158239808,
-0.0398656726,
-0.1532563865,
0.7825089693,
0.0298473723,
0.1465707272,
0.1259586662,
0.2123566121,
0.2996014655,
-0.3592542708,
-0.0047609629,
0.14062199,
0.3161544204,
-0.1753816456,
-0.4867202342,
0.3327391446,
0.0838660449,
0.1554297954,
-0.1178852171,
0.1441017538,
0.6216908693,
-0.1454122066,
0.5556603074,
0.0931106359,
0.0085586971,
-0.0513987988,
-0.3147474825,
0.3127994537,
0.4158589542,
0.347841382,
0.1352378279,
0.1462414265,
0.3830257952,
0.255897373,
-0.568416357,
-0.1476950347,
0.4132964015,
-0.1333667189,
-0.1575536281,
0.3834905326,
-0.0540320538,
0.1045508459,
0.0788131431,
0.1858241856,
0.0929148942,
0.375193119,
-0.0736511275,
0.2432726473,
0.2592487931,
0.0974980444,
0.3380096853,
0.2684294283,
-0.1351718456,
-0.3136456311,
-0.185309723,
0.0345402844,
0.123591125,
0.0863998383,
0.0447232239,
0.1819357574,
0.1531485468,
0.2662103474,
-0.0655694902,
0.1450532228,
-0.0084777437,
-0.0248161163,
-0.2228884995,
-0.1458397508,
0.1872135997,
-0.0870501548,
0.024511626,
-0.499504894,
-0.1176992208,
0.3334575295,
-0.2195060104,
-0.1744244248,
0.2647452056,
-0.3016047478,
0.0676542222,
-0.0331206918,
-0.1562540233,
-0.0631977916,
0.2823478281,
-0.361516118,
-0.2766749263,
0.2801630199,
-0.0707580298,
-0.3086366057,
0.4806893766,
0.3341833353,
0.5037378669,
-0.2653267086,
-0.1025480181,
-0.2228052467,
0.1971825212,
-0.1594049782,
0.2911326587,
-0.0202685036,
0.4730789065,
0.1639251709,
-0.1333217472,
0.5242288113,
0.0366240591,
0.0648770183,
0.5568506718,
-0.2519759834,
0.1873479784,
-0.2975605428,
-0.3276860714,
0.0573024079,
-0.1519959718,
0.4205704331,
0.1887064278,
-0.2505377233,
0.1852192134,
-0.0281150118,
0.3232849836,
-0.2908661366,
0.529887259,
-0.0202973038,
0.2953536212,
-0.0530070178,
0.0754796192,
-0.1315186024,
-0.1362609118,
-0.3146404326,
0.085203059,
-0.2904396057,
-0.0889269933,
-0.495698154,
-0.1259975731,
-0.0269061737,
0.1326653063,
-0.0939804465,
-0.1971267164,
-0.0234793276,
0.1047437191,
-0.0522618629,
0.2000953406,
0.0460238047,
0.2374133766,
0.1900678277,
0.2453594357,
-0.2602974772,
0.3165749609,
0.2897217274,
-0.1897236556,
-0.0601592809,
-0.361505717,
0.0874478891,
0.3728884161,
0.1571474671,
-0.5834988356,
-0.4987614453,
0.2187902927,
0.2269465625,
0.1632261872,
0.2620419264,
0.3271625042,
-0.2740885317,
-0.2698302567,
-0.5132446289,
-0.0430748798,
0.0780403614,
-0.1016154736,
-0.216723457
]
|
https://github.com/huggingface/datasets/issues/1965 | Can we parallelized the add_faiss_index process over dataset shards ? | @lhoestq As you mentioned faiss is already using multiprocessing. I tried to do the add_index with faiss for a dataset object inside a RAY actor and the process became very slow... if fact it takes so much time. It is because a ray actor comes with a single CPU core unless we assign it more. I also tried assigning more cores but still running add_index in the main process is very fast. | I am thinking of making the **add_faiss_index** process faster. What if we run the add_faiss_index process on separate dataset shards and then combine them before (dataset.concatenate) saving the faiss.index file ?
I feel theoretically this will reduce the accuracy of retrieval since it affects the indexing process.
@lhoestq
| 72 | Can we parallelized the add_faiss_index process over dataset shards ?
I am thinking of making the **add_faiss_index** process faster. What if we run the add_faiss_index process on separate dataset shards and then combine them before (dataset.concatenate) saving the faiss.index file ?
I feel theoretically this will reduce the accuracy of retrieval since it affects the indexing process.
@lhoestq
@lhoestq As you mentioned faiss is already using multiprocessing. I tried to do the add_index with faiss for a dataset object inside a RAY actor and the process became very slow... if fact it takes so much time. It is because a ray actor comes with a single CPU core unless we assign it more. I also tried assigning more cores but still running add_index in the main process is very fast. | [
-0.4054990709,
-0.1552562416,
-0.1257980019,
0.183027789,
-0.305123806,
0.2511135042,
0.3391771317,
0.0727011934,
0.1347900927,
0.1768684238,
-0.1435732991,
0.1960877925,
0.3504762053,
0.0879318342,
-0.2222459614,
0.0771599039,
0.3349465132,
-0.073750861,
0.1878648102,
0.1442666352,
-0.3492165804,
-0.079011701,
-0.0737033486,
-0.3046621978,
-0.2064708769,
0.2073305249,
-0.2225419134,
0.005088333,
0.1136126816,
-0.3396570981,
-0.2113554031,
0.296941638,
0.2204651386,
0.2022593617,
-0.0001236163,
-0.0930321738,
0.244729951,
0.1353628635,
0.2126867473,
0.7094952464,
-0.2860054374,
0.0842966884,
0.0118544279,
-0.2131094337,
0.0391237102,
-0.2245901525,
0.0313988589,
-0.3076616526,
-0.1945784986,
-0.2164340019,
0.0178864207,
-0.04201556,
0.1275666207,
0.0397085138,
-0.0555947907,
-0.2334745973,
-0.1960602552,
-0.1421742737,
0.1881549358,
0.4077613354,
0.0928777382,
0.1631822586,
-0.081511721,
-0.1110180989,
0.0442542769,
0.0191485696,
0.3126094043,
0.1118714362,
0.0620258674,
0.0274287388,
0.1938611269,
-0.1418396831,
-0.5114315748,
-0.1815448105,
0.1477665305,
-0.1431191564,
-0.1886235029,
-0.1851348579,
0.0634021163,
0.0095759816,
0.1191961244,
-0.4742817283,
0.0137951979,
-0.0145700639,
0.3781154156,
0.1848357767,
0.2552730739,
0.1527382284,
0.4181760252,
0.0640504286,
0.1389565021,
-0.0919337943,
-0.2201535106,
0.0968006924,
-0.7896096706,
0.0617333129,
-0.1457813233,
-0.1499474794,
-0.0559656397,
-0.1912729442,
-0.3814219534,
0.2947395146,
0.1131917238,
0.141847223,
0.0255720764,
-0.1043265536,
0.0632383823,
-0.2763234377,
-0.068123281,
-0.3750478327,
-0.0584924482,
0.0647540092,
0.0459295101,
-0.110150367,
-0.4853462279,
-0.0342457183,
-0.3445421457,
-0.1149134561,
-0.0397002101,
-0.2562004626,
0.1214182451,
-0.1795287728,
0.2044482231,
0.182708919,
0.3225756288,
0.1010045931,
-0.2328573316,
-0.001005588,
-0.0215396807,
-0.0223835912,
0.0048325537,
-0.1678573638,
0.1890093684,
0.4930633605,
-0.0246064235,
-0.4299057424,
-0.3655104637,
0.0114616398,
-0.0571342558,
0.1619333178,
0.0213698484,
-0.2168645263,
-0.0300495476,
0.0381839275,
-0.019889893,
-0.0655275658,
-0.1344531178,
0.2728835642,
-0.2350446433,
0.2151948661,
-0.2092876583,
-0.3914924562,
0.0174660124,
-0.0052476455,
-0.0216319393,
-0.1631600112,
-0.0698066503,
0.3777595162,
0.1984350383,
0.0365074649,
-0.0853618011,
0.1062018052,
-0.2503991425,
-0.1264425814,
0.2313386798,
0.1742166132,
-0.4121520817,
-0.0412241854,
-0.3235959709,
-0.1685477495,
0.1864795536,
0.4758312404,
-0.0292448569,
0.1094247922,
-0.333119601,
0.3905991912,
0.1770891398,
-0.3672056794,
0.0471230373,
0.1398413032,
-0.1204544753,
-0.1656449288,
0.331510663,
0.2888585031,
0.3453752398,
-0.0083531188,
0.135559395,
0.146589756,
-0.0612414107,
-0.1479461193,
-0.2127330601,
-0.2535598874,
0.16676566,
0.2950846255,
-0.0209491178,
0.1681787074,
-0.0310328826,
-0.8039102554,
0.3160692453,
-0.1459510475,
0.2999192476,
-0.0677222535,
0.2666431367,
0.3727968037,
0.3704650104,
-0.1162356883,
0.076976791,
0.2382862568,
0.0209096149,
-0.0338544287,
0.2227010727,
-0.3855348527,
0.4876060784,
0.0459408723,
0.0490641445,
0.4221504927,
-0.0552330203,
-0.1752132326,
0.0095420498,
-0.4162950516,
-0.3488957882,
0.5179666281,
-0.2398489416,
-0.184675619,
-0.069581002,
0.0604913421,
0.0085242083,
-0.1463851184,
-0.3137201965,
0.0875518918,
0.076388523,
-0.251897186,
-0.0870736316,
0.3075047135,
-0.0211860556,
0.0460117534,
0.8042545319,
0.0774964169,
-0.1413669437,
0.2354430407,
0.0844635218,
-0.2908228636,
-0.0603009909,
-0.1021530628,
0.3470937908,
0.1247009486,
0.1034971699,
0.4663465917,
0.0484495498,
-0.0102870613,
0.2089305818,
0.0494255982,
0.1752786785,
0.0404649079,
-0.0039794133,
-0.1105221212,
-0.0594567433,
0.0742546245,
-0.2332383394,
0.5649158359,
-0.1717336625,
-0.1027773693,
-0.2015343308,
-0.0461472645,
-0.0802181512,
0.147833094,
0.0841502622,
-0.0952416211,
0.2414818555,
0.2003470063,
0.030858973,
-0.2746875286,
0.2501147687,
-0.1929259449,
0.0255798344,
0.2707828581,
0.1545012295,
0.0391264707,
-0.0348358117,
-0.1922844499,
0.0270988271,
-0.1999225169,
0.0515194349,
0.1211282834,
-0.0166958869,
-0.1093713567,
0.0189683735,
0.1860829294,
-0.2127863914,
-0.1081466302,
-0.2143589556,
-0.1133391336,
0.1764409989,
0.0975931734,
-0.1917555332,
-0.0609497093,
-0.0961267948,
0.3963816762,
-0.1553370208,
-0.4528172314,
-0.2940586805,
-0.1470529437,
0.1323348135,
-0.0661902279,
0.3142539561,
0.085630931,
0.0921733975,
0.1264205426,
-0.040454112,
-0.3440810442,
0.0950985104,
-0.1518466622,
-0.0034037721,
-0.0941034034,
-0.2936230004,
-0.3489650786,
-0.181913048,
-0.0824744701,
0.0411472544,
0.0189426783,
0.0167010296,
-0.1476115137,
-0.1336667687,
-0.0711158589,
0.0677139759,
-0.1171518341,
-0.2093515694,
-0.3055463433,
0.3475597799,
-0.115881443,
0.1410504878,
-0.4759968817,
-0.1276792437,
-0.0629487336,
-0.3044451475,
-0.1956475526,
-0.2405064851,
-0.1052336916,
0.1640598923,
0.0499347262,
-0.1538970917,
0.0957069173,
0.0029417293,
-0.0289331414,
0.4696130753,
0.0203416627,
0.0658016577,
-0.0760874599,
0.1825040728,
-0.1649398655,
0.1721913964,
0.3239162862,
0.0056633838,
-0.0553415231,
0.1192135662,
0.117298536,
0.3369154036,
0.0030354538,
0.0329297036,
0.0064068167,
0.0400706604,
-0.2011461258,
0.7294511795,
0.1223026216,
-0.1957218349,
0.1946379989,
0.0824975073,
0.0250117164,
0.0861672163,
-0.1782758832,
0.3500839472,
0.1012572274,
-0.0929718167,
0.0026171785,
-0.0438821949,
-0.1264020503,
0.041188322,
-0.1293791234,
-0.1038939357,
-0.1315623075,
-0.054846365,
0.252861768,
0.5113690495,
-0.0436337031,
0.007744825,
-0.2366497815,
-0.0065445979,
0.1355664581,
0.4619988501,
0.5420898199,
-0.331613034,
0.0905429348,
-0.3064236343,
-0.3880170286,
0.4490928054,
0.0378731564,
0.4197947383,
0.084139742,
0.021097783,
0.2919822931,
-0.2219097316,
0.9026581049,
0.0270748027,
-0.5150842071,
0.0960619003,
-0.0259990916,
-0.331849128,
-0.0938114598,
0.1524904817,
0.2472437918,
0.3681123555,
0.1548549235,
-0.2120521814,
-0.2917937636,
0.1343270093,
0.0831082389,
-0.2784324586,
-0.673858285,
-0.149861902,
-0.0077253547,
-0.0534087345,
0.1820503175,
-0.2881645858,
0.1141938195,
0.0948081538,
-0.005959386,
-0.3301715851,
-0.023259148,
0.0441846475,
0.0040747891,
0.091508016,
-0.0205354486,
0.534684062,
0.2204899788,
-0.0735487193,
0.2417611629,
-0.0164078064,
0.2224722952,
0.145562008,
-0.1792442501,
-0.1392753869,
0.2092137337,
0.009841566,
-0.0030313171,
0.3491421044,
-0.2979665101,
0.1423682421,
-0.2083745003,
-0.0092652207,
0.0615615733,
0.4385182261,
-0.0940526202,
-0.2846192718,
0.3209878802,
0.1211754903,
-0.1498610377,
-0.1145357639,
0.3715473413,
-0.0501348525,
0.6364876628,
0.1283535957,
0.6783904433,
-0.2733450532,
-0.096475482,
-0.1757936031,
0.1353346407,
0.0775396824,
-0.5695589185,
0.407946378,
-0.2618126273,
-0.1729670912,
0.1151985899,
-0.1011654958,
0.1822105646,
0.267760396,
0.099764213,
0.2494497895,
0.0900089815,
-0.2832882702,
-0.1383252293,
0.3152261972,
0.1780464053,
-0.3854478002,
0.3567736745,
0.0085213063,
0.1185498461,
-0.2574541271,
0.0475541987,
0.0995274261,
-0.0964790955,
0.4011667669,
-0.1618816555,
-0.0622570813,
0.4725170732,
0.0784944817,
-0.1750521064,
0.0149901463,
0.2265221179,
-0.4040828645,
0.2418205887,
-0.2935245037,
-0.1323634237,
0.0944527164,
-0.0516432561,
-0.166447252,
0.0012743055,
-0.4851132631,
-0.0742492452,
0.0507861599,
-0.4548892379,
-0.1688658595,
0.2750448287,
-0.7210449576,
-0.3166677356,
0.0968270078,
-0.0611470155,
0.1004588157,
-0.1454279423,
0.382725507,
-0.134079963,
-0.0466317795,
-0.0264505167,
0.2520070076,
-0.4767292142,
0.8772203922,
-0.3962699473,
-0.0234913025,
0.0064208745,
0.2088802457,
0.3037535548,
-0.185749203,
0.0359903164,
0.0318610743,
-0.182581082,
-0.0297214519,
-0.7237133384,
-0.0615829267,
0.3477556705,
-0.1356629431,
0.0826941803,
-0.2126893103,
0.0430262797,
-0.5923836231,
-0.0986189172,
-0.0355290174,
-0.6348737478,
-0.0941626504,
-0.3619502783,
0.0876315683,
0.1204517633,
0.3645612597,
0.1034475341,
0.1033667326,
-0.347306639,
0.2193741202,
-0.145195812,
-0.0548932552,
0.2107296884,
0.1804766059,
0.2133189887,
-0.0586997345,
0.1368572116,
-0.0641076416,
-0.0119603528,
0.1239685416,
0.1416326314,
-0.0910777897,
0.2457413226,
0.2072181553,
0.0595081262,
0.0605388619,
-0.113494426,
-0.2933695316,
-0.258048594,
-0.2277738005,
0.0708862543,
-0.0550413765,
-0.2842024863,
-0.0373877585,
0.3796738684,
-0.2199349701,
-0.0367659144,
0.5711756945,
0.3638448119,
0.4119433165,
-0.1304622144,
0.5840528607,
-0.1503132582,
-0.0116173718,
0.1101325527,
0.038004797,
0.3578720093,
0.1273036152,
0.0257703848,
0.1005512848,
-0.2617843747,
-0.2099004984,
0.5314102769,
0.513040781,
0.4117484093,
-0.2642949224,
0.0969006941,
0.0436327606,
-0.0635159984,
-0.1413561404,
0.7605379224,
-0.0652893633,
0.2589676678,
0.2289000899,
0.3385690749,
0.3246197402,
-0.2439426482,
0.0713544786,
0.1840889156,
0.3953976333,
-0.2592019439,
-0.5113337636,
0.3347236812,
0.0319293961,
0.2751270831,
-0.1516728848,
0.1107718125,
0.923040092,
-0.1979515254,
0.5783289671,
0.269702673,
0.0731895119,
0.0477504805,
-0.1127757803,
0.5054670572,
0.2119086236,
0.2594750226,
0.0425580367,
0.1568236798,
0.4555433393,
0.086291492,
-0.4791411757,
-0.203067854,
0.3633429706,
-0.0651319697,
-0.1690252721,
0.3964123428,
0.0012901124,
0.0932266712,
0.0458044447,
0.2178767323,
0.0357575789,
0.3246456087,
0.0491058864,
0.1516366452,
0.1504165977,
0.0357428603,
0.4240094125,
0.2561798394,
0.0196467489,
-0.2027693242,
-0.102305375,
-0.0331464484,
0.1279640198,
0.168108359,
0.0743427873,
0.1605705172,
0.0622820109,
0.3273923993,
-0.0757853985,
0.1349638849,
-0.0273563936,
-0.0791053772,
-0.3827724159,
-0.0394778512,
0.1916843057,
-0.0211961046,
-0.0130573139,
-0.4874781072,
-0.2010088265,
0.362059325,
-0.0939693376,
-0.0635305792,
0.1349420547,
-0.3062335849,
0.0034933316,
0.0126203066,
-0.1436278075,
-0.1309952885,
0.3259010911,
-0.5958312154,
-0.2522016764,
0.1412501484,
-0.021780977,
-0.2656866908,
0.4139504731,
0.0934202299,
0.5814161897,
-0.2157099247,
-0.018151639,
-0.298273623,
0.1779897064,
-0.1417058259,
0.0568242855,
-0.0309726652,
0.473413676,
-0.0127441892,
-0.1003620997,
0.640220046,
0.0816159919,
0.2973897755,
0.5808255076,
-0.2128430754,
0.1888260245,
-0.2031278312,
-0.2613909543,
0.1296496093,
-0.1679708362,
0.4393238425,
0.165476948,
-0.1756121963,
0.1502399594,
-0.1133836582,
0.42543751,
-0.4664508104,
0.4777958393,
0.0649800748,
0.2579564154,
-0.1346857697,
0.1684642881,
-0.132851854,
-0.1170496494,
-0.3317904174,
-0.0999454707,
-0.2547703683,
-0.1374462098,
-0.3246657848,
-0.1422875226,
-0.0342454091,
0.3217969537,
-0.157991901,
-0.0471630432,
-0.127691105,
0.1993936449,
-0.1976647675,
0.1830365062,
-0.0548232235,
0.160077855,
0.2074938715,
0.1919040382,
-0.2801052332,
0.2080819011,
0.2239716351,
-0.2235784531,
-0.0168866105,
-0.4630414248,
0.1518836766,
0.3087670505,
0.1494834125,
-0.4369867444,
-0.440608412,
0.0744837075,
0.2929987609,
0.1704964638,
0.194023788,
0.0582562387,
-0.2841021121,
-0.261177361,
-0.5086883903,
0.0182645954,
0.1092124134,
-0.0496228337,
-0.0873969793
]
|
https://github.com/huggingface/datasets/issues/1964 | Datasets.py function load_dataset does not match squad dataset | Hi !
To fix 1, an you try to run this code ?
```python
from datasets import load_dataset
load_dataset("squad", download_mode="force_redownload")
```
Maybe the file your downloaded was corrupted, in this case redownloading this way should fix your issue 1.
Regarding your 2nd point, you're right that loading the raw json this way doesn't give you a dataset with the column "context", "question" and "answers". Indeed the squad format is a very nested format so you have to preprocess the data. You can do it this way:
```python
def process_squad(examples):
"""
Process a dataset in the squad format with columns "title" and "paragraphs"
to return the dataset with columns "context", "question" and "answers".
"""
out = {"context": [], "question": [], "answers":[]}
for paragraphs in examples["paragraphs"]:
for paragraph in paragraphs:
for qa in paragraph["qas"]:
answers = [{"answer_start": answer["answer_start"], "text": answer["text"].strip()} for answer in qa["answers"]]
out["context"].append(paragraph["context"].strip())
out["question"].append(qa["question"].strip())
out["answers"].append(answers)
return out
datasets = load_dataset(extension, data_files=data_files, field="data")
column_names = datasets["train"].column_names
if set(column_names) == {"title", "paragraphs"}:
datasets = datasets.map(process_squad, batched=True, remove_columns=column_names)
```
Hope that helps :) | ### 1 When I try to train lxmert,and follow the code in README that --dataset name:
```shell
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --dataset_name squad --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
the bug is that:
```
Downloading and preparing dataset squad/plain_text (download: 33.51 MiB, generated: 85.75 MiB, post-processed: Unknown size, total: 119.27 MiB) to /home2/zhenggo1/.cache/huggingface/datasets/squad/plain_text/1.0.0/4c81550d83a2ac7c7ce23783bd8ff36642800e6633c1f18417fb58c3ff50cdd7...
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 217, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/load.py", line 746, in load_dataset
use_auth_token=use_auth_token,
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 573, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 633, in _download_and_prepare
self.info.download_checksums, dl_manager.get_recorded_sizes_checksums(), "dataset source files"
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/utils/info_utils.py", line 39, in verify_checksums
raise NonMatchingChecksumError(error_msg + str(bad_urls))
datasets.utils.info_utils.NonMatchingChecksumError: Checksums didn't match for dataset source files:
['https://rajpurkar.github.io/SQuAD-explorer/dataset/train-v1.1.json']
```
And I try to find the [checksum link](https://github.com/huggingface/datasets/blob/master/datasets/squad/dataset_infos.json)
,is the problem plain_text do not have a checksum?
### 2 When I try to train lxmert,and use local dataset:
```
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --train_file $SQUAD_DIR/train-v1.1.json --validation_file $SQUAD_DIR/dev-v1.1.json --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
The bug is that
```
['title', 'paragraphs']
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 273, in main
answer_column_name = "answers" if "answers" in column_names else column_names[2]
IndexError: list index out of range
```
I print the answer_column_name and find that local squad dataset need the package datasets to preprocessing so that the code below can work:
```
if training_args.do_train:
column_names = datasets["train"].column_names
else:
column_names = datasets["validation"].column_names
print(datasets["train"].column_names)
question_column_name = "question" if "question" in column_names else column_names[0]
context_column_name = "context" if "context" in column_names else column_names[1]
answer_column_name = "answers" if "answers" in column_names else column_names[2]
```
## Please tell me how to fix the bug,thks a lot! | 170 | Datasets.py function load_dataset does not match squad dataset
### 1 When I try to train lxmert,and follow the code in README that --dataset name:
```shell
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --dataset_name squad --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
the bug is that:
```
Downloading and preparing dataset squad/plain_text (download: 33.51 MiB, generated: 85.75 MiB, post-processed: Unknown size, total: 119.27 MiB) to /home2/zhenggo1/.cache/huggingface/datasets/squad/plain_text/1.0.0/4c81550d83a2ac7c7ce23783bd8ff36642800e6633c1f18417fb58c3ff50cdd7...
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 217, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/load.py", line 746, in load_dataset
use_auth_token=use_auth_token,
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 573, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 633, in _download_and_prepare
self.info.download_checksums, dl_manager.get_recorded_sizes_checksums(), "dataset source files"
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/utils/info_utils.py", line 39, in verify_checksums
raise NonMatchingChecksumError(error_msg + str(bad_urls))
datasets.utils.info_utils.NonMatchingChecksumError: Checksums didn't match for dataset source files:
['https://rajpurkar.github.io/SQuAD-explorer/dataset/train-v1.1.json']
```
And I try to find the [checksum link](https://github.com/huggingface/datasets/blob/master/datasets/squad/dataset_infos.json)
,is the problem plain_text do not have a checksum?
### 2 When I try to train lxmert,and use local dataset:
```
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --train_file $SQUAD_DIR/train-v1.1.json --validation_file $SQUAD_DIR/dev-v1.1.json --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
The bug is that
```
['title', 'paragraphs']
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 273, in main
answer_column_name = "answers" if "answers" in column_names else column_names[2]
IndexError: list index out of range
```
I print the answer_column_name and find that local squad dataset need the package datasets to preprocessing so that the code below can work:
```
if training_args.do_train:
column_names = datasets["train"].column_names
else:
column_names = datasets["validation"].column_names
print(datasets["train"].column_names)
question_column_name = "question" if "question" in column_names else column_names[0]
context_column_name = "context" if "context" in column_names else column_names[1]
answer_column_name = "answers" if "answers" in column_names else column_names[2]
```
## Please tell me how to fix the bug,thks a lot!
Hi !
To fix 1, an you try to run this code ?
```python
from datasets import load_dataset
load_dataset("squad", download_mode="force_redownload")
```
Maybe the file your downloaded was corrupted, in this case redownloading this way should fix your issue 1.
Regarding your 2nd point, you're right that loading the raw json this way doesn't give you a dataset with the column "context", "question" and "answers". Indeed the squad format is a very nested format so you have to preprocess the data. You can do it this way:
```python
def process_squad(examples):
"""
Process a dataset in the squad format with columns "title" and "paragraphs"
to return the dataset with columns "context", "question" and "answers".
"""
out = {"context": [], "question": [], "answers":[]}
for paragraphs in examples["paragraphs"]:
for paragraph in paragraphs:
for qa in paragraph["qas"]:
answers = [{"answer_start": answer["answer_start"], "text": answer["text"].strip()} for answer in qa["answers"]]
out["context"].append(paragraph["context"].strip())
out["question"].append(qa["question"].strip())
out["answers"].append(answers)
return out
datasets = load_dataset(extension, data_files=data_files, field="data")
column_names = datasets["train"].column_names
if set(column_names) == {"title", "paragraphs"}:
datasets = datasets.map(process_squad, batched=True, remove_columns=column_names)
```
Hope that helps :) | [
-0.353720516,
0.062508136,
0.0333653875,
0.3963990211,
0.5554528236,
0.0057210471,
0.5457585454,
0.3404690027,
-0.0983098298,
-0.1216232702,
-0.1650660038,
0.4508371055,
0.1084151044,
-0.0774194002,
0.1859293282,
0.2271615863,
-0.1242240593,
-0.0123368576,
-0.146108225,
-0.2893434763,
-0.1109782234,
0.1282913834,
-0.0823142305,
0.2603986561,
-0.1788989604,
0.0166908335,
0.2006343305,
0.272364527,
-0.0037961234,
-0.2199689448,
0.3428422213,
-0.2606053054,
0.2361017913,
0.5758854747,
-0.0001148581,
0.1132559404,
-0.0115429182,
-0.3397287428,
-0.5327041745,
-0.200222224,
-0.1021838337,
-0.3757724166,
0.1486450732,
-0.2605550587,
-0.1595322788,
0.0937372819,
0.0203168094,
-0.4645365775,
0.3544548452,
0.4883139133,
0.2139826268,
0.2522563934,
-0.1001007184,
0.0775510296,
0.1142543554,
-0.0009760716,
-0.0695089027,
0.1360990107,
0.334751159,
-0.0582687482,
0.0025544665,
0.1724458039,
0.0828082114,
0.0568235703,
0.2703093886,
0.00640395,
0.1991152614,
-0.316155225,
0.0564420745,
0.3177836835,
0.4627766013,
-0.4630154967,
-0.3347049356,
-0.2631345689,
0.1331043243,
-0.2565730214,
0.132016629,
0.0672779083,
0.0511588715,
-0.0424275659,
0.0477761962,
0.0376647562,
0.1114289686,
0.0368288346,
0.0068917884,
0.1999943256,
0.0288671236,
0.2383964211,
-0.2015398741,
-0.113548398,
0.1148922071,
-0.0831490755,
0.081831947,
0.3924934268,
-0.8285178542,
-0.0814113691,
-0.204295367,
-0.1421722323,
0.2562874258,
0.0819008574,
0.0386919901,
-0.0269713923,
0.1486689448,
0.1044572815,
0.1687799692,
0.3692039847,
0.3052024841,
0.2674018741,
-0.0663637966,
0.0668205172,
-0.3717198074,
0.2254105806,
-0.3225814104,
-0.1618057787,
0.0960094184,
0.0835883096,
0.0062301527,
-0.1927383393,
-0.5517168641,
0.1761803031,
0.1544087827,
0.0026421717,
0.097437188,
0.3247915506,
0.0759161711,
0.3802294135,
0.0263225865,
0.10485553,
-0.2946235538,
-0.3704166412,
-0.2408784926,
0.0330557562,
-0.088010259,
0.0268362667,
0.0183389839,
-0.0356005244,
0.3227836788,
-0.1957619935,
0.2114949375,
-0.1790798306,
0.1150043458,
-0.2556710541,
-0.1285900921,
-0.0065956297,
0.029810125,
0.0322049223,
0.1742972434,
-0.3730716109,
-0.0482526384,
0.1222927868,
-0.3712682724,
-0.033652354,
-0.1820592135,
0.187116161,
-0.1391082406,
0.0208787471,
-0.2373691797,
0.1698869169,
0.3073554337,
-0.1878398061,
0.1063958183,
-0.3961227238,
-0.1664003581,
-0.2122270465,
0.3151643872,
0.3882878423,
-0.407456696,
-0.2117101997,
0.1249394417,
-0.0952139422,
-0.0381637625,
0.1216654554,
-0.1516054869,
0.1555612236,
-0.2062239051,
-0.0070254449,
0.7145841718,
-0.8443274498,
-0.4243375659,
0.1099731028,
-0.3059237003,
-0.0194986593,
-0.0710132122,
0.0664077923,
0.1930086017,
0.0147336926,
0.0827302188,
0.5066138506,
-0.0362929925,
0.0914033353,
-0.1724579334,
-0.2712132037,
0.2526646852,
0.1708514988,
0.0318142623,
-0.0380644612,
0.0388375632,
0.4989835918,
0.332213819,
0.1426165998,
0.1112047285,
-0.0299586449,
-0.035939917,
-0.0821635276,
0.1146057397,
-0.1481087208,
-0.6798555851,
0.163865611,
-0.1644513905,
0.0439886115,
0.241078347,
-0.1508303434,
-0.2135134488,
-0.0917366818,
-0.4252308309,
-0.1023876891,
0.0743928775,
0.1495984048,
-0.0585199855,
-0.1226448193,
-0.1598846763,
0.3514792919,
-0.2154511809,
0.2284578085,
-0.4849060178,
-0.0965390131,
-0.0637454763,
-0.0362155698,
0.0537011735,
0.3318409622,
0.0871920362,
-0.0709578618,
-0.1057533845,
0.4979955852,
0.0180258043,
0.1023962423,
0.2883630097,
-0.2016446739,
0.0996332839,
-0.0990449861,
0.0020645163,
0.2259094566,
0.1946659386,
-0.0812614039,
0.0224433318,
0.1418917328,
0.1574392319,
0.0746555328,
-0.004860986,
-0.1536110938,
0.1390525997,
0.0118647087,
0.0111015849,
0.0971625224,
0.0520382561,
0.2940090001,
0.5483118296,
0.1111360267,
-0.1868931949,
0.1310920715,
0.3315557837,
-0.0650450736,
0.275726229,
-0.0043976414,
-0.1713227183,
-0.0783392489,
0.101006113,
0.1789459586,
0.5017950535,
-0.0036269187,
-0.1863487959,
-0.1349208206,
-0.06367746,
-0.0468236953,
0.0711337999,
-0.1824290454,
0.2553721368,
0.2577781379,
0.1839799583,
0.0547975227,
-0.1531580985,
-0.049743522,
0.0207578279,
0.2905255556,
-0.3741136193,
0.0461584181,
-0.1272042692,
0.0979504138,
-0.3777190149,
0.133810699,
-0.3502557576,
-0.1833579242,
-0.13573125,
-0.1108107418,
0.3093049228,
0.2101664692,
-0.3485353291,
0.1555946469,
0.2402639687,
-0.5443776846,
0.1576042026,
0.1009901762,
-0.3830035925,
0.0529526398,
0.1205425113,
-0.069621712,
-0.0969109163,
-0.1528278291,
0.0173261613,
-0.120101966,
-0.168003723,
0.1868709624,
-0.0529081486,
0.576834023,
-0.0123321833,
0.17038773,
-0.0075948229,
-0.0680121183,
0.1667571813,
-0.1843082756,
-0.1167619526,
-0.1657408476,
-0.1121703982,
0.0364884101,
-0.0776864365,
-0.622492671,
-0.4673991501,
-0.298817575,
-0.1805041134,
0.0007812897,
0.2649473846,
0.0936489254,
0.0102783879,
0.3407399356,
-0.0355759561,
0.0361260287,
-0.4035872817,
-0.2818410397,
0.2556054592,
0.0628467649,
-0.2947934866,
-0.0994649306,
0.0628204867,
0.2072018534,
-0.0956360623,
-0.3958401084,
-0.2739954591,
0.2307889462,
-0.0112172971,
-0.0910095274,
0.0472383387,
0.115157485,
-0.1613558531,
0.1061526015,
-0.2067127079,
-0.5061610341,
0.1450342834,
0.1470153183,
0.6205662489,
-0.1660098732,
0.2980053425,
-0.0526382141,
0.6775238514,
0.1890773475,
-0.22801736,
0.2107889056,
-0.2105232626,
0.3032868505,
0.0035383345,
-0.2769272923,
0.303943038,
0.0719058588,
0.0459163487,
0.3248113692,
0.0534217097,
-0.0166757554,
-0.0613192208,
0.3597290814,
-0.1392308474,
-0.1571272016,
-0.0605639182,
-0.0752927959,
-0.270031482,
0.0741318464,
0.3065281808,
-0.1772013009,
-0.167300418,
-0.1517760158,
0.4453921914,
-0.0859651268,
0.2577698827,
-0.6791189909,
-0.0043503684,
-0.1259898245,
0.2868065238,
-0.1449967921,
0.6176646352,
-0.0544952974,
-0.1857381314,
-0.0650188327,
0.0791010112,
0.4798837006,
-0.0459978692,
-0.0093407119,
-0.0361170769,
0.2946528494,
-0.6179637909,
-0.1878313869,
0.177603066,
0.168530792,
0.0936853215,
0.3528565466,
-0.1821607947,
-0.0771108866,
0.1613402218,
0.1991808563,
-0.1662609726,
-0.0170081481,
-0.3835246861,
-0.2073012292,
-0.3429888785,
-0.0511816852,
-0.2764179111,
0.1328060031,
0.1391487271,
0.1173347309,
0.2389437258,
-0.1128841564,
0.3738931715,
0.1367883533,
0.3835943937,
0.1973971426,
0.1353372186,
0.2334741801,
0.2617396414,
-0.1502474099,
0.4892303944,
-0.4564042687,
-0.300064683,
0.0343395285,
-0.0737036765,
0.1476983726,
0.3775241375,
-0.1436185092,
0.0837648883,
-0.3207883835,
-0.0862554684,
0.2754157186,
0.1629421562,
0.2510093153,
0.0771015659,
-0.1133953929,
-0.4098264277,
0.1798561662,
-0.1120343506,
-0.0426193736,
0.2145652324,
-0.4636404514,
-0.2888950408,
0.0964141116,
-0.1039727405,
0.8939821124,
-0.0649744123,
0.1889764816,
0.3377414048,
0.163262412,
0.282250911,
-0.0774448365,
0.1654772758,
-0.4589557946,
-0.0782303363,
0.0744743645,
-0.1392125636,
-0.0647865608,
0.3663624823,
0.0141664529,
0.5146530271,
-0.2688011825,
0.3116568029,
0.0540140532,
0.5210407972,
-0.1337995678,
-0.0108749662,
-0.3472366631,
0.1949187219,
-0.1809045076,
0.2802455127,
-0.120389536,
0.0054682009,
-0.3029420078,
-0.1379411668,
-0.1319408566,
0.2274258137,
-0.5376274586,
0.1669766158,
0.0934275612,
-0.348642379,
0.0191773418,
0.5643285513,
-0.1002913713,
-0.0820769295,
-0.1084555537,
0.3530824482,
0.0207635928,
0.3046308756,
0.0768878311,
0.045780655,
0.4243013263,
-0.0168561283,
-0.0948248357,
0.0720395148,
-0.0042468379,
-0.3094083369,
-0.2512998581,
0.0408150665,
0.3062187433,
-0.275687933,
-0.1972320974,
-0.1188385338,
0.0159462802,
-0.0596126914,
0.1198261082,
0.0611224659,
-0.0966570675,
0.2068723142,
0.1296120584,
-0.4074322283,
0.0401295908,
0.3414309621,
0.0581959039,
0.0030539334,
0.6670225859,
0.0741894022,
0.0704763979,
-0.1949027628,
0.1497740597,
0.3011733294,
-0.2336236238,
-0.0392005332,
-0.200078547,
-0.2490166873,
0.0004849647,
-0.1210952103,
0.2407470047,
-0.0176704507,
0.0402800739,
-0.4578700364,
-0.4342665076,
0.0315098315,
-0.0630611703,
0.0092654163,
0.0782027319,
-0.0439873487,
0.14506495,
0.0453661829,
-0.2561044991,
0.2107703835,
-0.1966145635,
0.2120199651,
0.0993525088,
-0.040149983,
0.2497250438,
-0.0228080787,
0.0503341965,
-0.0272153113,
-0.3081597984,
-0.1973446459,
-0.1295123994,
0.1182671338,
0.0220860038,
-0.0156389251,
-0.1738800704,
-0.2943679392,
-0.1543197334,
-0.2474768013,
-0.0346161574,
0.0881622881,
0.0274492465,
0.014234297,
-0.0534724034,
0.0265386216,
0.065787822,
-0.0739741847,
0.0375888459,
0.0440007299,
0.2452985197,
0.1012573615,
0.0479806922,
-0.0090397513,
-0.5060155988,
0.1013092324,
0.1878548414,
0.3835131526,
0.0299074557,
-0.1631865799,
-0.0384694189,
0.2729741931,
0.0583595075,
0.4147218466,
-0.4566238821,
-0.0442087837,
-0.0886463895,
0.1434324086,
-0.217178762,
0.0970661119,
0.3625799417,
-0.1833088547,
-0.0155576682,
0.2735647261,
0.1266647428,
0.1897180825,
0.0217409395,
0.106899038,
0.5871457458,
-0.0874020159,
0.1646407843,
0.2900646031,
-0.2160798907,
0.2205363363,
0.3349782526,
-0.0837545395,
-0.0087307738,
0.3994483054,
-0.1929350495,
0.3431118131,
-0.0790345296,
-0.1280988157,
0.2440440208,
-0.2488020658,
0.0799285248,
0.4024313986,
-0.1566055417,
0.1699330956,
0.046969384,
0.5395813584,
-0.4212686121,
-0.1374427974,
-0.0382388309,
-0.1659312993,
-0.1484350711,
-0.0598561019,
0.0648373216,
-0.1156119779,
-0.1001708359,
-0.2388542593,
-0.1484496295,
-0.3574168086,
-0.0503092706,
-0.0039663431,
-0.3478865921,
-0.0714052841,
-0.068698734,
0.3550386727,
-0.1964207739,
-0.1058575436,
0.3332425058,
-0.1416483521,
0.0370898917,
0.3267585635,
0.2570814192,
0.1953930259,
0.445263952,
0.0781016648,
-0.1183170602,
0.3155587018,
0.0447257794,
0.0146261593,
0.1478068382,
-0.1330284029,
-0.197568655,
0.3923157454,
0.1372192055,
-0.0548008978,
-0.2756022513,
0.3441490829,
-0.06653478,
-0.2472363561,
0.2151203603,
-0.2440630496,
-0.0380831659,
-0.5316841006,
0.3191307783,
-0.3130172789,
0.0980826393,
0.4218283296,
0.1543096006,
0.3799420595,
-0.2579368949,
0.0727035925,
-0.0288272742,
0.3897283077,
0.1729899645,
-0.2461687028,
-0.1826698333,
0.1881108582,
-0.9209600091,
0.4458394349,
0.0589725003,
-0.0390838832,
0.0334377028,
0.343989253,
0.053749647,
0.053144753,
0.2887403071,
-0.0962834731,
0.1624651998,
-0.0415592231,
-0.3789628446,
-0.3341311216,
-0.3106013238,
0.4049323201,
0.1494320631,
-0.5924780369,
0.2898758352,
0.0384889767,
-0.0501694456,
-0.1049866974,
-0.2132234871,
0.1590007693,
-0.1347790956,
0.4179120064,
-0.1402911395,
0.4938714206,
-0.0273788664,
-0.0191060323,
-0.2152628452,
-0.1111689806,
-0.295769453,
0.2690995634,
-0.1461154521,
0.0767934695,
0.1251029819,
0.0760324299,
-0.2987894714,
0.3234612346,
0.1469840556,
-0.2812111974,
-0.4066530764,
-0.021511659,
0.037754409,
0.0922570676,
0.0601234101,
0.3981384039,
-0.1498903483,
0.2334182113,
-0.1931377202,
-0.3373350799,
0.5052193403,
-0.3257140219,
-0.1926413476,
-0.274181962,
0.2520014048,
0.1326460689,
0.0052114832,
-0.6400470734,
0.0537734814,
0.3680358827,
-0.1895974576,
-0.1688361317,
0.1406752467,
-0.234420985,
0.4075177908,
0.0046435916,
0.0375307649,
0.0067872964,
-0.130524829,
0.1636308432,
-0.2550604045
]
|
https://github.com/huggingface/datasets/issues/1964 | Datasets.py function load_dataset does not match squad dataset | Thks for quickly answering!
### 1 I try the first way,but seems not work
```
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 503, in <module>
main()
File "examples/question-answering/run_qa.py", line 218, in main
datasets = load_dataset(data_args.dataset_name, download_mode="force_redownload")
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/load.py", line 746, in load_dataset
use_auth_token=use_auth_token,
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 573, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 633, in _download_and_prepare
self.info.download_checksums, dl_manager.get_recorded_sizes_checksums(), "dataset source files"
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/utils/info_utils.py", line 39, in verify_checksums
raise NonMatchingChecksumError(error_msg + str(bad_urls))
datasets.utils.info_utils.NonMatchingChecksumError: Checksums didn't match for dataset source files:
['https://rajpurkar.github.io/SQuAD-explorer/dataset/train-v1.1.json']
```
### 2 I try the second way,and run the examples/question-answering/run_qa.py,it lead to another bug orz..
```
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 523, in <module>
main()
File "examples/question-answering/run_qa.py", line 379, in main
load_from_cache_file=not data_args.overwrite_cache,
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/arrow_dataset.py", line 1120, in map
update_data = does_function_return_dict(test_inputs, test_indices)
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/arrow_dataset.py", line 1091, in does_function_return_dict
function(*fn_args, indices, **fn_kwargs) if with_indices else function(*fn_args, **fn_kwargs)
File "examples/question-answering/run_qa.py", line 339, in prepare_train_features
if len(answers["answer_start"]) == 0:
TypeError: list indices must be integers or slices, not str
```
## may be the function prepare_train_features in run_qa.py need to fix,I think is that the prep
```python
for i, offsets in enumerate(offset_mapping):
# We will label impossible answers with the index of the CLS token.
input_ids = tokenized_examples["input_ids"][i]
cls_index = input_ids.index(tokenizer.cls_token_id)
# Grab the sequence corresponding to that example (to know what is the context and what is the question).
sequence_ids = tokenized_examples.sequence_ids(i)
# One example can give several spans, this is the index of the example containing this span of text.
sample_index = sample_mapping[i]
answers = examples[answer_column_name][sample_index]
print(examples,answers)
# If no answers are given, set the cls_index as answer.
if len(answers["answer_start"]) == 0:
tokenized_examples["start_positions"].append(cls_index)
tokenized_examples["end_positions"].append(cls_index)
else:
# Start/end character index of the answer in the text.
start_char = answers["answer_start"][0]
end_char = start_char + len(answers["text"][0])
# Start token index of the current span in the text.
token_start_index = 0
while sequence_ids[token_start_index] != (1 if pad_on_right else 0):
token_start_index += 1
# End token index of the current span in the text.
token_end_index = len(input_ids) - 1
while sequence_ids[token_end_index] != (1 if pad_on_right else 0):
token_end_index -= 1
# Detect if the answer is out of the span (in which case this feature is labeled with the CLS index).
if not (offsets[token_start_index][0] <= start_char and offsets[token_end_index][1] >= end_char):
tokenized_examples["start_positions"].append(cls_index)
tokenized_examples["end_positions"].append(cls_index)
else:
# Otherwise move the token_start_index and token_end_index to the two ends of the answer.
# Note: we could go after the last offset if the answer is the last word (edge case).
while token_start_index < len(offsets) and offsets[token_start_index][0] <= start_char:
token_start_index += 1
tokenized_examples["start_positions"].append(token_start_index - 1)
while offsets[token_end_index][1] >= end_char:
token_end_index -= 1
tokenized_examples["end_positions"].append(token_end_index + 1)
return tokenized_examples
``` | ### 1 When I try to train lxmert,and follow the code in README that --dataset name:
```shell
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --dataset_name squad --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
the bug is that:
```
Downloading and preparing dataset squad/plain_text (download: 33.51 MiB, generated: 85.75 MiB, post-processed: Unknown size, total: 119.27 MiB) to /home2/zhenggo1/.cache/huggingface/datasets/squad/plain_text/1.0.0/4c81550d83a2ac7c7ce23783bd8ff36642800e6633c1f18417fb58c3ff50cdd7...
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 217, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/load.py", line 746, in load_dataset
use_auth_token=use_auth_token,
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 573, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 633, in _download_and_prepare
self.info.download_checksums, dl_manager.get_recorded_sizes_checksums(), "dataset source files"
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/utils/info_utils.py", line 39, in verify_checksums
raise NonMatchingChecksumError(error_msg + str(bad_urls))
datasets.utils.info_utils.NonMatchingChecksumError: Checksums didn't match for dataset source files:
['https://rajpurkar.github.io/SQuAD-explorer/dataset/train-v1.1.json']
```
And I try to find the [checksum link](https://github.com/huggingface/datasets/blob/master/datasets/squad/dataset_infos.json)
,is the problem plain_text do not have a checksum?
### 2 When I try to train lxmert,and use local dataset:
```
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --train_file $SQUAD_DIR/train-v1.1.json --validation_file $SQUAD_DIR/dev-v1.1.json --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
The bug is that
```
['title', 'paragraphs']
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 273, in main
answer_column_name = "answers" if "answers" in column_names else column_names[2]
IndexError: list index out of range
```
I print the answer_column_name and find that local squad dataset need the package datasets to preprocessing so that the code below can work:
```
if training_args.do_train:
column_names = datasets["train"].column_names
else:
column_names = datasets["validation"].column_names
print(datasets["train"].column_names)
question_column_name = "question" if "question" in column_names else column_names[0]
context_column_name = "context" if "context" in column_names else column_names[1]
answer_column_name = "answers" if "answers" in column_names else column_names[2]
```
## Please tell me how to fix the bug,thks a lot! | 434 | Datasets.py function load_dataset does not match squad dataset
### 1 When I try to train lxmert,and follow the code in README that --dataset name:
```shell
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --dataset_name squad --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
the bug is that:
```
Downloading and preparing dataset squad/plain_text (download: 33.51 MiB, generated: 85.75 MiB, post-processed: Unknown size, total: 119.27 MiB) to /home2/zhenggo1/.cache/huggingface/datasets/squad/plain_text/1.0.0/4c81550d83a2ac7c7ce23783bd8ff36642800e6633c1f18417fb58c3ff50cdd7...
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 217, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/load.py", line 746, in load_dataset
use_auth_token=use_auth_token,
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 573, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 633, in _download_and_prepare
self.info.download_checksums, dl_manager.get_recorded_sizes_checksums(), "dataset source files"
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/utils/info_utils.py", line 39, in verify_checksums
raise NonMatchingChecksumError(error_msg + str(bad_urls))
datasets.utils.info_utils.NonMatchingChecksumError: Checksums didn't match for dataset source files:
['https://rajpurkar.github.io/SQuAD-explorer/dataset/train-v1.1.json']
```
And I try to find the [checksum link](https://github.com/huggingface/datasets/blob/master/datasets/squad/dataset_infos.json)
,is the problem plain_text do not have a checksum?
### 2 When I try to train lxmert,and use local dataset:
```
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --train_file $SQUAD_DIR/train-v1.1.json --validation_file $SQUAD_DIR/dev-v1.1.json --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
The bug is that
```
['title', 'paragraphs']
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 273, in main
answer_column_name = "answers" if "answers" in column_names else column_names[2]
IndexError: list index out of range
```
I print the answer_column_name and find that local squad dataset need the package datasets to preprocessing so that the code below can work:
```
if training_args.do_train:
column_names = datasets["train"].column_names
else:
column_names = datasets["validation"].column_names
print(datasets["train"].column_names)
question_column_name = "question" if "question" in column_names else column_names[0]
context_column_name = "context" if "context" in column_names else column_names[1]
answer_column_name = "answers" if "answers" in column_names else column_names[2]
```
## Please tell me how to fix the bug,thks a lot!
Thks for quickly answering!
### 1 I try the first way,but seems not work
```
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 503, in <module>
main()
File "examples/question-answering/run_qa.py", line 218, in main
datasets = load_dataset(data_args.dataset_name, download_mode="force_redownload")
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/load.py", line 746, in load_dataset
use_auth_token=use_auth_token,
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 573, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 633, in _download_and_prepare
self.info.download_checksums, dl_manager.get_recorded_sizes_checksums(), "dataset source files"
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/utils/info_utils.py", line 39, in verify_checksums
raise NonMatchingChecksumError(error_msg + str(bad_urls))
datasets.utils.info_utils.NonMatchingChecksumError: Checksums didn't match for dataset source files:
['https://rajpurkar.github.io/SQuAD-explorer/dataset/train-v1.1.json']
```
### 2 I try the second way,and run the examples/question-answering/run_qa.py,it lead to another bug orz..
```
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 523, in <module>
main()
File "examples/question-answering/run_qa.py", line 379, in main
load_from_cache_file=not data_args.overwrite_cache,
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/arrow_dataset.py", line 1120, in map
update_data = does_function_return_dict(test_inputs, test_indices)
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/arrow_dataset.py", line 1091, in does_function_return_dict
function(*fn_args, indices, **fn_kwargs) if with_indices else function(*fn_args, **fn_kwargs)
File "examples/question-answering/run_qa.py", line 339, in prepare_train_features
if len(answers["answer_start"]) == 0:
TypeError: list indices must be integers or slices, not str
```
## may be the function prepare_train_features in run_qa.py need to fix,I think is that the prep
```python
for i, offsets in enumerate(offset_mapping):
# We will label impossible answers with the index of the CLS token.
input_ids = tokenized_examples["input_ids"][i]
cls_index = input_ids.index(tokenizer.cls_token_id)
# Grab the sequence corresponding to that example (to know what is the context and what is the question).
sequence_ids = tokenized_examples.sequence_ids(i)
# One example can give several spans, this is the index of the example containing this span of text.
sample_index = sample_mapping[i]
answers = examples[answer_column_name][sample_index]
print(examples,answers)
# If no answers are given, set the cls_index as answer.
if len(answers["answer_start"]) == 0:
tokenized_examples["start_positions"].append(cls_index)
tokenized_examples["end_positions"].append(cls_index)
else:
# Start/end character index of the answer in the text.
start_char = answers["answer_start"][0]
end_char = start_char + len(answers["text"][0])
# Start token index of the current span in the text.
token_start_index = 0
while sequence_ids[token_start_index] != (1 if pad_on_right else 0):
token_start_index += 1
# End token index of the current span in the text.
token_end_index = len(input_ids) - 1
while sequence_ids[token_end_index] != (1 if pad_on_right else 0):
token_end_index -= 1
# Detect if the answer is out of the span (in which case this feature is labeled with the CLS index).
if not (offsets[token_start_index][0] <= start_char and offsets[token_end_index][1] >= end_char):
tokenized_examples["start_positions"].append(cls_index)
tokenized_examples["end_positions"].append(cls_index)
else:
# Otherwise move the token_start_index and token_end_index to the two ends of the answer.
# Note: we could go after the last offset if the answer is the last word (edge case).
while token_start_index < len(offsets) and offsets[token_start_index][0] <= start_char:
token_start_index += 1
tokenized_examples["start_positions"].append(token_start_index - 1)
while offsets[token_end_index][1] >= end_char:
token_end_index -= 1
tokenized_examples["end_positions"].append(token_end_index + 1)
return tokenized_examples
``` | [
-0.353720516,
0.062508136,
0.0333653875,
0.3963990211,
0.5554528236,
0.0057210471,
0.5457585454,
0.3404690027,
-0.0983098298,
-0.1216232702,
-0.1650660038,
0.4508371055,
0.1084151044,
-0.0774194002,
0.1859293282,
0.2271615863,
-0.1242240593,
-0.0123368576,
-0.146108225,
-0.2893434763,
-0.1109782234,
0.1282913834,
-0.0823142305,
0.2603986561,
-0.1788989604,
0.0166908335,
0.2006343305,
0.272364527,
-0.0037961234,
-0.2199689448,
0.3428422213,
-0.2606053054,
0.2361017913,
0.5758854747,
-0.0001148581,
0.1132559404,
-0.0115429182,
-0.3397287428,
-0.5327041745,
-0.200222224,
-0.1021838337,
-0.3757724166,
0.1486450732,
-0.2605550587,
-0.1595322788,
0.0937372819,
0.0203168094,
-0.4645365775,
0.3544548452,
0.4883139133,
0.2139826268,
0.2522563934,
-0.1001007184,
0.0775510296,
0.1142543554,
-0.0009760716,
-0.0695089027,
0.1360990107,
0.334751159,
-0.0582687482,
0.0025544665,
0.1724458039,
0.0828082114,
0.0568235703,
0.2703093886,
0.00640395,
0.1991152614,
-0.316155225,
0.0564420745,
0.3177836835,
0.4627766013,
-0.4630154967,
-0.3347049356,
-0.2631345689,
0.1331043243,
-0.2565730214,
0.132016629,
0.0672779083,
0.0511588715,
-0.0424275659,
0.0477761962,
0.0376647562,
0.1114289686,
0.0368288346,
0.0068917884,
0.1999943256,
0.0288671236,
0.2383964211,
-0.2015398741,
-0.113548398,
0.1148922071,
-0.0831490755,
0.081831947,
0.3924934268,
-0.8285178542,
-0.0814113691,
-0.204295367,
-0.1421722323,
0.2562874258,
0.0819008574,
0.0386919901,
-0.0269713923,
0.1486689448,
0.1044572815,
0.1687799692,
0.3692039847,
0.3052024841,
0.2674018741,
-0.0663637966,
0.0668205172,
-0.3717198074,
0.2254105806,
-0.3225814104,
-0.1618057787,
0.0960094184,
0.0835883096,
0.0062301527,
-0.1927383393,
-0.5517168641,
0.1761803031,
0.1544087827,
0.0026421717,
0.097437188,
0.3247915506,
0.0759161711,
0.3802294135,
0.0263225865,
0.10485553,
-0.2946235538,
-0.3704166412,
-0.2408784926,
0.0330557562,
-0.088010259,
0.0268362667,
0.0183389839,
-0.0356005244,
0.3227836788,
-0.1957619935,
0.2114949375,
-0.1790798306,
0.1150043458,
-0.2556710541,
-0.1285900921,
-0.0065956297,
0.029810125,
0.0322049223,
0.1742972434,
-0.3730716109,
-0.0482526384,
0.1222927868,
-0.3712682724,
-0.033652354,
-0.1820592135,
0.187116161,
-0.1391082406,
0.0208787471,
-0.2373691797,
0.1698869169,
0.3073554337,
-0.1878398061,
0.1063958183,
-0.3961227238,
-0.1664003581,
-0.2122270465,
0.3151643872,
0.3882878423,
-0.407456696,
-0.2117101997,
0.1249394417,
-0.0952139422,
-0.0381637625,
0.1216654554,
-0.1516054869,
0.1555612236,
-0.2062239051,
-0.0070254449,
0.7145841718,
-0.8443274498,
-0.4243375659,
0.1099731028,
-0.3059237003,
-0.0194986593,
-0.0710132122,
0.0664077923,
0.1930086017,
0.0147336926,
0.0827302188,
0.5066138506,
-0.0362929925,
0.0914033353,
-0.1724579334,
-0.2712132037,
0.2526646852,
0.1708514988,
0.0318142623,
-0.0380644612,
0.0388375632,
0.4989835918,
0.332213819,
0.1426165998,
0.1112047285,
-0.0299586449,
-0.035939917,
-0.0821635276,
0.1146057397,
-0.1481087208,
-0.6798555851,
0.163865611,
-0.1644513905,
0.0439886115,
0.241078347,
-0.1508303434,
-0.2135134488,
-0.0917366818,
-0.4252308309,
-0.1023876891,
0.0743928775,
0.1495984048,
-0.0585199855,
-0.1226448193,
-0.1598846763,
0.3514792919,
-0.2154511809,
0.2284578085,
-0.4849060178,
-0.0965390131,
-0.0637454763,
-0.0362155698,
0.0537011735,
0.3318409622,
0.0871920362,
-0.0709578618,
-0.1057533845,
0.4979955852,
0.0180258043,
0.1023962423,
0.2883630097,
-0.2016446739,
0.0996332839,
-0.0990449861,
0.0020645163,
0.2259094566,
0.1946659386,
-0.0812614039,
0.0224433318,
0.1418917328,
0.1574392319,
0.0746555328,
-0.004860986,
-0.1536110938,
0.1390525997,
0.0118647087,
0.0111015849,
0.0971625224,
0.0520382561,
0.2940090001,
0.5483118296,
0.1111360267,
-0.1868931949,
0.1310920715,
0.3315557837,
-0.0650450736,
0.275726229,
-0.0043976414,
-0.1713227183,
-0.0783392489,
0.101006113,
0.1789459586,
0.5017950535,
-0.0036269187,
-0.1863487959,
-0.1349208206,
-0.06367746,
-0.0468236953,
0.0711337999,
-0.1824290454,
0.2553721368,
0.2577781379,
0.1839799583,
0.0547975227,
-0.1531580985,
-0.049743522,
0.0207578279,
0.2905255556,
-0.3741136193,
0.0461584181,
-0.1272042692,
0.0979504138,
-0.3777190149,
0.133810699,
-0.3502557576,
-0.1833579242,
-0.13573125,
-0.1108107418,
0.3093049228,
0.2101664692,
-0.3485353291,
0.1555946469,
0.2402639687,
-0.5443776846,
0.1576042026,
0.1009901762,
-0.3830035925,
0.0529526398,
0.1205425113,
-0.069621712,
-0.0969109163,
-0.1528278291,
0.0173261613,
-0.120101966,
-0.168003723,
0.1868709624,
-0.0529081486,
0.576834023,
-0.0123321833,
0.17038773,
-0.0075948229,
-0.0680121183,
0.1667571813,
-0.1843082756,
-0.1167619526,
-0.1657408476,
-0.1121703982,
0.0364884101,
-0.0776864365,
-0.622492671,
-0.4673991501,
-0.298817575,
-0.1805041134,
0.0007812897,
0.2649473846,
0.0936489254,
0.0102783879,
0.3407399356,
-0.0355759561,
0.0361260287,
-0.4035872817,
-0.2818410397,
0.2556054592,
0.0628467649,
-0.2947934866,
-0.0994649306,
0.0628204867,
0.2072018534,
-0.0956360623,
-0.3958401084,
-0.2739954591,
0.2307889462,
-0.0112172971,
-0.0910095274,
0.0472383387,
0.115157485,
-0.1613558531,
0.1061526015,
-0.2067127079,
-0.5061610341,
0.1450342834,
0.1470153183,
0.6205662489,
-0.1660098732,
0.2980053425,
-0.0526382141,
0.6775238514,
0.1890773475,
-0.22801736,
0.2107889056,
-0.2105232626,
0.3032868505,
0.0035383345,
-0.2769272923,
0.303943038,
0.0719058588,
0.0459163487,
0.3248113692,
0.0534217097,
-0.0166757554,
-0.0613192208,
0.3597290814,
-0.1392308474,
-0.1571272016,
-0.0605639182,
-0.0752927959,
-0.270031482,
0.0741318464,
0.3065281808,
-0.1772013009,
-0.167300418,
-0.1517760158,
0.4453921914,
-0.0859651268,
0.2577698827,
-0.6791189909,
-0.0043503684,
-0.1259898245,
0.2868065238,
-0.1449967921,
0.6176646352,
-0.0544952974,
-0.1857381314,
-0.0650188327,
0.0791010112,
0.4798837006,
-0.0459978692,
-0.0093407119,
-0.0361170769,
0.2946528494,
-0.6179637909,
-0.1878313869,
0.177603066,
0.168530792,
0.0936853215,
0.3528565466,
-0.1821607947,
-0.0771108866,
0.1613402218,
0.1991808563,
-0.1662609726,
-0.0170081481,
-0.3835246861,
-0.2073012292,
-0.3429888785,
-0.0511816852,
-0.2764179111,
0.1328060031,
0.1391487271,
0.1173347309,
0.2389437258,
-0.1128841564,
0.3738931715,
0.1367883533,
0.3835943937,
0.1973971426,
0.1353372186,
0.2334741801,
0.2617396414,
-0.1502474099,
0.4892303944,
-0.4564042687,
-0.300064683,
0.0343395285,
-0.0737036765,
0.1476983726,
0.3775241375,
-0.1436185092,
0.0837648883,
-0.3207883835,
-0.0862554684,
0.2754157186,
0.1629421562,
0.2510093153,
0.0771015659,
-0.1133953929,
-0.4098264277,
0.1798561662,
-0.1120343506,
-0.0426193736,
0.2145652324,
-0.4636404514,
-0.2888950408,
0.0964141116,
-0.1039727405,
0.8939821124,
-0.0649744123,
0.1889764816,
0.3377414048,
0.163262412,
0.282250911,
-0.0774448365,
0.1654772758,
-0.4589557946,
-0.0782303363,
0.0744743645,
-0.1392125636,
-0.0647865608,
0.3663624823,
0.0141664529,
0.5146530271,
-0.2688011825,
0.3116568029,
0.0540140532,
0.5210407972,
-0.1337995678,
-0.0108749662,
-0.3472366631,
0.1949187219,
-0.1809045076,
0.2802455127,
-0.120389536,
0.0054682009,
-0.3029420078,
-0.1379411668,
-0.1319408566,
0.2274258137,
-0.5376274586,
0.1669766158,
0.0934275612,
-0.348642379,
0.0191773418,
0.5643285513,
-0.1002913713,
-0.0820769295,
-0.1084555537,
0.3530824482,
0.0207635928,
0.3046308756,
0.0768878311,
0.045780655,
0.4243013263,
-0.0168561283,
-0.0948248357,
0.0720395148,
-0.0042468379,
-0.3094083369,
-0.2512998581,
0.0408150665,
0.3062187433,
-0.275687933,
-0.1972320974,
-0.1188385338,
0.0159462802,
-0.0596126914,
0.1198261082,
0.0611224659,
-0.0966570675,
0.2068723142,
0.1296120584,
-0.4074322283,
0.0401295908,
0.3414309621,
0.0581959039,
0.0030539334,
0.6670225859,
0.0741894022,
0.0704763979,
-0.1949027628,
0.1497740597,
0.3011733294,
-0.2336236238,
-0.0392005332,
-0.200078547,
-0.2490166873,
0.0004849647,
-0.1210952103,
0.2407470047,
-0.0176704507,
0.0402800739,
-0.4578700364,
-0.4342665076,
0.0315098315,
-0.0630611703,
0.0092654163,
0.0782027319,
-0.0439873487,
0.14506495,
0.0453661829,
-0.2561044991,
0.2107703835,
-0.1966145635,
0.2120199651,
0.0993525088,
-0.040149983,
0.2497250438,
-0.0228080787,
0.0503341965,
-0.0272153113,
-0.3081597984,
-0.1973446459,
-0.1295123994,
0.1182671338,
0.0220860038,
-0.0156389251,
-0.1738800704,
-0.2943679392,
-0.1543197334,
-0.2474768013,
-0.0346161574,
0.0881622881,
0.0274492465,
0.014234297,
-0.0534724034,
0.0265386216,
0.065787822,
-0.0739741847,
0.0375888459,
0.0440007299,
0.2452985197,
0.1012573615,
0.0479806922,
-0.0090397513,
-0.5060155988,
0.1013092324,
0.1878548414,
0.3835131526,
0.0299074557,
-0.1631865799,
-0.0384694189,
0.2729741931,
0.0583595075,
0.4147218466,
-0.4566238821,
-0.0442087837,
-0.0886463895,
0.1434324086,
-0.217178762,
0.0970661119,
0.3625799417,
-0.1833088547,
-0.0155576682,
0.2735647261,
0.1266647428,
0.1897180825,
0.0217409395,
0.106899038,
0.5871457458,
-0.0874020159,
0.1646407843,
0.2900646031,
-0.2160798907,
0.2205363363,
0.3349782526,
-0.0837545395,
-0.0087307738,
0.3994483054,
-0.1929350495,
0.3431118131,
-0.0790345296,
-0.1280988157,
0.2440440208,
-0.2488020658,
0.0799285248,
0.4024313986,
-0.1566055417,
0.1699330956,
0.046969384,
0.5395813584,
-0.4212686121,
-0.1374427974,
-0.0382388309,
-0.1659312993,
-0.1484350711,
-0.0598561019,
0.0648373216,
-0.1156119779,
-0.1001708359,
-0.2388542593,
-0.1484496295,
-0.3574168086,
-0.0503092706,
-0.0039663431,
-0.3478865921,
-0.0714052841,
-0.068698734,
0.3550386727,
-0.1964207739,
-0.1058575436,
0.3332425058,
-0.1416483521,
0.0370898917,
0.3267585635,
0.2570814192,
0.1953930259,
0.445263952,
0.0781016648,
-0.1183170602,
0.3155587018,
0.0447257794,
0.0146261593,
0.1478068382,
-0.1330284029,
-0.197568655,
0.3923157454,
0.1372192055,
-0.0548008978,
-0.2756022513,
0.3441490829,
-0.06653478,
-0.2472363561,
0.2151203603,
-0.2440630496,
-0.0380831659,
-0.5316841006,
0.3191307783,
-0.3130172789,
0.0980826393,
0.4218283296,
0.1543096006,
0.3799420595,
-0.2579368949,
0.0727035925,
-0.0288272742,
0.3897283077,
0.1729899645,
-0.2461687028,
-0.1826698333,
0.1881108582,
-0.9209600091,
0.4458394349,
0.0589725003,
-0.0390838832,
0.0334377028,
0.343989253,
0.053749647,
0.053144753,
0.2887403071,
-0.0962834731,
0.1624651998,
-0.0415592231,
-0.3789628446,
-0.3341311216,
-0.3106013238,
0.4049323201,
0.1494320631,
-0.5924780369,
0.2898758352,
0.0384889767,
-0.0501694456,
-0.1049866974,
-0.2132234871,
0.1590007693,
-0.1347790956,
0.4179120064,
-0.1402911395,
0.4938714206,
-0.0273788664,
-0.0191060323,
-0.2152628452,
-0.1111689806,
-0.295769453,
0.2690995634,
-0.1461154521,
0.0767934695,
0.1251029819,
0.0760324299,
-0.2987894714,
0.3234612346,
0.1469840556,
-0.2812111974,
-0.4066530764,
-0.021511659,
0.037754409,
0.0922570676,
0.0601234101,
0.3981384039,
-0.1498903483,
0.2334182113,
-0.1931377202,
-0.3373350799,
0.5052193403,
-0.3257140219,
-0.1926413476,
-0.274181962,
0.2520014048,
0.1326460689,
0.0052114832,
-0.6400470734,
0.0537734814,
0.3680358827,
-0.1895974576,
-0.1688361317,
0.1406752467,
-0.234420985,
0.4075177908,
0.0046435916,
0.0375307649,
0.0067872964,
-0.130524829,
0.1636308432,
-0.2550604045
]
|
https://github.com/huggingface/datasets/issues/1964 | Datasets.py function load_dataset does not match squad dataset | ## I have fixed it, @lhoestq
### the first section change as you said and add ["id"]
```python
def process_squad(examples):
"""
Process a dataset in the squad format with columns "title" and "paragraphs"
to return the dataset with columns "context", "question" and "answers".
"""
# print(examples)
out = {"context": [], "question": [], "answers":[],"id":[]}
for paragraphs in examples["paragraphs"]:
for paragraph in paragraphs:
for qa in paragraph["qas"]:
answers = [{"answer_start": answer["answer_start"], "text": answer["text"].strip()} for answer in qa["answers"]]
out["context"].append(paragraph["context"].strip())
out["question"].append(qa["question"].strip())
out["answers"].append(answers)
out["id"].append(qa["id"])
return out
column_names = datasets["train"].column_names if training_args.do_train else datasets["validation"].column_names
# print(datasets["train"].column_names)
if set(column_names) == {"title", "paragraphs"}:
datasets = datasets.map(process_squad, batched=True, remove_columns=column_names)
# Preprocessing the datasets.
# Preprocessing is slighlty different for training and evaluation.
if training_args.do_train:
column_names = datasets["train"].column_names
else:
column_names = datasets["validation"].column_names
# print(column_names)
question_column_name = "question" if "question" in column_names else column_names[0]
context_column_name = "context" if "context" in column_names else column_names[1]
answer_column_name = "answers" if "answers" in column_names else column_names[2]
```
### the second section
```python
def prepare_train_features(examples):
# Tokenize our examples with truncation and maybe padding, but keep the overflows using a stride. This results
# in one example possible giving several features when a context is long, each of those features having a
# context that overlaps a bit the context of the previous feature.
tokenized_examples = tokenizer(
examples[question_column_name if pad_on_right else context_column_name],
examples[context_column_name if pad_on_right else question_column_name],
truncation="only_second" if pad_on_right else "only_first",
max_length=data_args.max_seq_length,
stride=data_args.doc_stride,
return_overflowing_tokens=True,
return_offsets_mapping=True,
padding="max_length" if data_args.pad_to_max_length else False,
)
# Since one example might give us several features if it has a long context, we need a map from a feature to
# its corresponding example. This key gives us just that.
sample_mapping = tokenized_examples.pop("overflow_to_sample_mapping")
# The offset mappings will give us a map from token to character position in the original context. This will
# help us compute the start_positions and end_positions.
offset_mapping = tokenized_examples.pop("offset_mapping")
# Let's label those examples!
tokenized_examples["start_positions"] = []
tokenized_examples["end_positions"] = []
for i, offsets in enumerate(offset_mapping):
# We will label impossible answers with the index of the CLS token.
input_ids = tokenized_examples["input_ids"][i]
cls_index = input_ids.index(tokenizer.cls_token_id)
# Grab the sequence corresponding to that example (to know what is the context and what is the question).
sequence_ids = tokenized_examples.sequence_ids(i)
# One example can give several spans, this is the index of the example containing this span of text.
sample_index = sample_mapping[i]
answers = examples[answer_column_name][sample_index]
# print(examples,answers,offset_mapping,tokenized_examples)
# If no answers are given, set the cls_index as answer.
if len(answers) == 0:#len(answers["answer_start"]) == 0:
tokenized_examples["start_positions"].append(cls_index)
tokenized_examples["end_positions"].append(cls_index)
else:
# Start/end character index of the answer in the text.
start_char = answers[0]["answer_start"]
end_char = start_char + len(answers[0]["text"])
# Start token index of the current span in the text.
token_start_index = 0
while sequence_ids[token_start_index] != (1 if pad_on_right else 0):
token_start_index += 1
# End token index of the current span in the text.
token_end_index = len(input_ids) - 1
while sequence_ids[token_end_index] != (1 if pad_on_right else 0):
token_end_index -= 1
# Detect if the answer is out of the span (in which case this feature is labeled with the CLS index).
if not (offsets[token_start_index][0] <= start_char and offsets[token_end_index][1] >= end_char):
tokenized_examples["start_positions"].append(cls_index)
tokenized_examples["end_positions"].append(cls_index)
else:
# Otherwise move the token_start_index and token_end_index to the two ends of the answer.
# Note: we could go after the last offset if the answer is the last word (edge case).
while token_start_index < len(offsets) and offsets[token_start_index][0] <= start_char:
token_start_index += 1
tokenized_examples["start_positions"].append(token_start_index - 1)
while offsets[token_end_index][1] >= end_char:
token_end_index -= 1
tokenized_examples["end_positions"].append(token_end_index + 1)
return tokenized_examples
``` | ### 1 When I try to train lxmert,and follow the code in README that --dataset name:
```shell
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --dataset_name squad --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
the bug is that:
```
Downloading and preparing dataset squad/plain_text (download: 33.51 MiB, generated: 85.75 MiB, post-processed: Unknown size, total: 119.27 MiB) to /home2/zhenggo1/.cache/huggingface/datasets/squad/plain_text/1.0.0/4c81550d83a2ac7c7ce23783bd8ff36642800e6633c1f18417fb58c3ff50cdd7...
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 217, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/load.py", line 746, in load_dataset
use_auth_token=use_auth_token,
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 573, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 633, in _download_and_prepare
self.info.download_checksums, dl_manager.get_recorded_sizes_checksums(), "dataset source files"
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/utils/info_utils.py", line 39, in verify_checksums
raise NonMatchingChecksumError(error_msg + str(bad_urls))
datasets.utils.info_utils.NonMatchingChecksumError: Checksums didn't match for dataset source files:
['https://rajpurkar.github.io/SQuAD-explorer/dataset/train-v1.1.json']
```
And I try to find the [checksum link](https://github.com/huggingface/datasets/blob/master/datasets/squad/dataset_infos.json)
,is the problem plain_text do not have a checksum?
### 2 When I try to train lxmert,and use local dataset:
```
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --train_file $SQUAD_DIR/train-v1.1.json --validation_file $SQUAD_DIR/dev-v1.1.json --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
The bug is that
```
['title', 'paragraphs']
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 273, in main
answer_column_name = "answers" if "answers" in column_names else column_names[2]
IndexError: list index out of range
```
I print the answer_column_name and find that local squad dataset need the package datasets to preprocessing so that the code below can work:
```
if training_args.do_train:
column_names = datasets["train"].column_names
else:
column_names = datasets["validation"].column_names
print(datasets["train"].column_names)
question_column_name = "question" if "question" in column_names else column_names[0]
context_column_name = "context" if "context" in column_names else column_names[1]
answer_column_name = "answers" if "answers" in column_names else column_names[2]
```
## Please tell me how to fix the bug,thks a lot! | 569 | Datasets.py function load_dataset does not match squad dataset
### 1 When I try to train lxmert,and follow the code in README that --dataset name:
```shell
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --dataset_name squad --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
the bug is that:
```
Downloading and preparing dataset squad/plain_text (download: 33.51 MiB, generated: 85.75 MiB, post-processed: Unknown size, total: 119.27 MiB) to /home2/zhenggo1/.cache/huggingface/datasets/squad/plain_text/1.0.0/4c81550d83a2ac7c7ce23783bd8ff36642800e6633c1f18417fb58c3ff50cdd7...
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 217, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/load.py", line 746, in load_dataset
use_auth_token=use_auth_token,
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 573, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 633, in _download_and_prepare
self.info.download_checksums, dl_manager.get_recorded_sizes_checksums(), "dataset source files"
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/utils/info_utils.py", line 39, in verify_checksums
raise NonMatchingChecksumError(error_msg + str(bad_urls))
datasets.utils.info_utils.NonMatchingChecksumError: Checksums didn't match for dataset source files:
['https://rajpurkar.github.io/SQuAD-explorer/dataset/train-v1.1.json']
```
And I try to find the [checksum link](https://github.com/huggingface/datasets/blob/master/datasets/squad/dataset_infos.json)
,is the problem plain_text do not have a checksum?
### 2 When I try to train lxmert,and use local dataset:
```
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --train_file $SQUAD_DIR/train-v1.1.json --validation_file $SQUAD_DIR/dev-v1.1.json --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
The bug is that
```
['title', 'paragraphs']
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 273, in main
answer_column_name = "answers" if "answers" in column_names else column_names[2]
IndexError: list index out of range
```
I print the answer_column_name and find that local squad dataset need the package datasets to preprocessing so that the code below can work:
```
if training_args.do_train:
column_names = datasets["train"].column_names
else:
column_names = datasets["validation"].column_names
print(datasets["train"].column_names)
question_column_name = "question" if "question" in column_names else column_names[0]
context_column_name = "context" if "context" in column_names else column_names[1]
answer_column_name = "answers" if "answers" in column_names else column_names[2]
```
## Please tell me how to fix the bug,thks a lot!
## I have fixed it, @lhoestq
### the first section change as you said and add ["id"]
```python
def process_squad(examples):
"""
Process a dataset in the squad format with columns "title" and "paragraphs"
to return the dataset with columns "context", "question" and "answers".
"""
# print(examples)
out = {"context": [], "question": [], "answers":[],"id":[]}
for paragraphs in examples["paragraphs"]:
for paragraph in paragraphs:
for qa in paragraph["qas"]:
answers = [{"answer_start": answer["answer_start"], "text": answer["text"].strip()} for answer in qa["answers"]]
out["context"].append(paragraph["context"].strip())
out["question"].append(qa["question"].strip())
out["answers"].append(answers)
out["id"].append(qa["id"])
return out
column_names = datasets["train"].column_names if training_args.do_train else datasets["validation"].column_names
# print(datasets["train"].column_names)
if set(column_names) == {"title", "paragraphs"}:
datasets = datasets.map(process_squad, batched=True, remove_columns=column_names)
# Preprocessing the datasets.
# Preprocessing is slighlty different for training and evaluation.
if training_args.do_train:
column_names = datasets["train"].column_names
else:
column_names = datasets["validation"].column_names
# print(column_names)
question_column_name = "question" if "question" in column_names else column_names[0]
context_column_name = "context" if "context" in column_names else column_names[1]
answer_column_name = "answers" if "answers" in column_names else column_names[2]
```
### the second section
```python
def prepare_train_features(examples):
# Tokenize our examples with truncation and maybe padding, but keep the overflows using a stride. This results
# in one example possible giving several features when a context is long, each of those features having a
# context that overlaps a bit the context of the previous feature.
tokenized_examples = tokenizer(
examples[question_column_name if pad_on_right else context_column_name],
examples[context_column_name if pad_on_right else question_column_name],
truncation="only_second" if pad_on_right else "only_first",
max_length=data_args.max_seq_length,
stride=data_args.doc_stride,
return_overflowing_tokens=True,
return_offsets_mapping=True,
padding="max_length" if data_args.pad_to_max_length else False,
)
# Since one example might give us several features if it has a long context, we need a map from a feature to
# its corresponding example. This key gives us just that.
sample_mapping = tokenized_examples.pop("overflow_to_sample_mapping")
# The offset mappings will give us a map from token to character position in the original context. This will
# help us compute the start_positions and end_positions.
offset_mapping = tokenized_examples.pop("offset_mapping")
# Let's label those examples!
tokenized_examples["start_positions"] = []
tokenized_examples["end_positions"] = []
for i, offsets in enumerate(offset_mapping):
# We will label impossible answers with the index of the CLS token.
input_ids = tokenized_examples["input_ids"][i]
cls_index = input_ids.index(tokenizer.cls_token_id)
# Grab the sequence corresponding to that example (to know what is the context and what is the question).
sequence_ids = tokenized_examples.sequence_ids(i)
# One example can give several spans, this is the index of the example containing this span of text.
sample_index = sample_mapping[i]
answers = examples[answer_column_name][sample_index]
# print(examples,answers,offset_mapping,tokenized_examples)
# If no answers are given, set the cls_index as answer.
if len(answers) == 0:#len(answers["answer_start"]) == 0:
tokenized_examples["start_positions"].append(cls_index)
tokenized_examples["end_positions"].append(cls_index)
else:
# Start/end character index of the answer in the text.
start_char = answers[0]["answer_start"]
end_char = start_char + len(answers[0]["text"])
# Start token index of the current span in the text.
token_start_index = 0
while sequence_ids[token_start_index] != (1 if pad_on_right else 0):
token_start_index += 1
# End token index of the current span in the text.
token_end_index = len(input_ids) - 1
while sequence_ids[token_end_index] != (1 if pad_on_right else 0):
token_end_index -= 1
# Detect if the answer is out of the span (in which case this feature is labeled with the CLS index).
if not (offsets[token_start_index][0] <= start_char and offsets[token_end_index][1] >= end_char):
tokenized_examples["start_positions"].append(cls_index)
tokenized_examples["end_positions"].append(cls_index)
else:
# Otherwise move the token_start_index and token_end_index to the two ends of the answer.
# Note: we could go after the last offset if the answer is the last word (edge case).
while token_start_index < len(offsets) and offsets[token_start_index][0] <= start_char:
token_start_index += 1
tokenized_examples["start_positions"].append(token_start_index - 1)
while offsets[token_end_index][1] >= end_char:
token_end_index -= 1
tokenized_examples["end_positions"].append(token_end_index + 1)
return tokenized_examples
``` | [
-0.353720516,
0.062508136,
0.0333653875,
0.3963990211,
0.5554528236,
0.0057210471,
0.5457585454,
0.3404690027,
-0.0983098298,
-0.1216232702,
-0.1650660038,
0.4508371055,
0.1084151044,
-0.0774194002,
0.1859293282,
0.2271615863,
-0.1242240593,
-0.0123368576,
-0.146108225,
-0.2893434763,
-0.1109782234,
0.1282913834,
-0.0823142305,
0.2603986561,
-0.1788989604,
0.0166908335,
0.2006343305,
0.272364527,
-0.0037961234,
-0.2199689448,
0.3428422213,
-0.2606053054,
0.2361017913,
0.5758854747,
-0.0001148581,
0.1132559404,
-0.0115429182,
-0.3397287428,
-0.5327041745,
-0.200222224,
-0.1021838337,
-0.3757724166,
0.1486450732,
-0.2605550587,
-0.1595322788,
0.0937372819,
0.0203168094,
-0.4645365775,
0.3544548452,
0.4883139133,
0.2139826268,
0.2522563934,
-0.1001007184,
0.0775510296,
0.1142543554,
-0.0009760716,
-0.0695089027,
0.1360990107,
0.334751159,
-0.0582687482,
0.0025544665,
0.1724458039,
0.0828082114,
0.0568235703,
0.2703093886,
0.00640395,
0.1991152614,
-0.316155225,
0.0564420745,
0.3177836835,
0.4627766013,
-0.4630154967,
-0.3347049356,
-0.2631345689,
0.1331043243,
-0.2565730214,
0.132016629,
0.0672779083,
0.0511588715,
-0.0424275659,
0.0477761962,
0.0376647562,
0.1114289686,
0.0368288346,
0.0068917884,
0.1999943256,
0.0288671236,
0.2383964211,
-0.2015398741,
-0.113548398,
0.1148922071,
-0.0831490755,
0.081831947,
0.3924934268,
-0.8285178542,
-0.0814113691,
-0.204295367,
-0.1421722323,
0.2562874258,
0.0819008574,
0.0386919901,
-0.0269713923,
0.1486689448,
0.1044572815,
0.1687799692,
0.3692039847,
0.3052024841,
0.2674018741,
-0.0663637966,
0.0668205172,
-0.3717198074,
0.2254105806,
-0.3225814104,
-0.1618057787,
0.0960094184,
0.0835883096,
0.0062301527,
-0.1927383393,
-0.5517168641,
0.1761803031,
0.1544087827,
0.0026421717,
0.097437188,
0.3247915506,
0.0759161711,
0.3802294135,
0.0263225865,
0.10485553,
-0.2946235538,
-0.3704166412,
-0.2408784926,
0.0330557562,
-0.088010259,
0.0268362667,
0.0183389839,
-0.0356005244,
0.3227836788,
-0.1957619935,
0.2114949375,
-0.1790798306,
0.1150043458,
-0.2556710541,
-0.1285900921,
-0.0065956297,
0.029810125,
0.0322049223,
0.1742972434,
-0.3730716109,
-0.0482526384,
0.1222927868,
-0.3712682724,
-0.033652354,
-0.1820592135,
0.187116161,
-0.1391082406,
0.0208787471,
-0.2373691797,
0.1698869169,
0.3073554337,
-0.1878398061,
0.1063958183,
-0.3961227238,
-0.1664003581,
-0.2122270465,
0.3151643872,
0.3882878423,
-0.407456696,
-0.2117101997,
0.1249394417,
-0.0952139422,
-0.0381637625,
0.1216654554,
-0.1516054869,
0.1555612236,
-0.2062239051,
-0.0070254449,
0.7145841718,
-0.8443274498,
-0.4243375659,
0.1099731028,
-0.3059237003,
-0.0194986593,
-0.0710132122,
0.0664077923,
0.1930086017,
0.0147336926,
0.0827302188,
0.5066138506,
-0.0362929925,
0.0914033353,
-0.1724579334,
-0.2712132037,
0.2526646852,
0.1708514988,
0.0318142623,
-0.0380644612,
0.0388375632,
0.4989835918,
0.332213819,
0.1426165998,
0.1112047285,
-0.0299586449,
-0.035939917,
-0.0821635276,
0.1146057397,
-0.1481087208,
-0.6798555851,
0.163865611,
-0.1644513905,
0.0439886115,
0.241078347,
-0.1508303434,
-0.2135134488,
-0.0917366818,
-0.4252308309,
-0.1023876891,
0.0743928775,
0.1495984048,
-0.0585199855,
-0.1226448193,
-0.1598846763,
0.3514792919,
-0.2154511809,
0.2284578085,
-0.4849060178,
-0.0965390131,
-0.0637454763,
-0.0362155698,
0.0537011735,
0.3318409622,
0.0871920362,
-0.0709578618,
-0.1057533845,
0.4979955852,
0.0180258043,
0.1023962423,
0.2883630097,
-0.2016446739,
0.0996332839,
-0.0990449861,
0.0020645163,
0.2259094566,
0.1946659386,
-0.0812614039,
0.0224433318,
0.1418917328,
0.1574392319,
0.0746555328,
-0.004860986,
-0.1536110938,
0.1390525997,
0.0118647087,
0.0111015849,
0.0971625224,
0.0520382561,
0.2940090001,
0.5483118296,
0.1111360267,
-0.1868931949,
0.1310920715,
0.3315557837,
-0.0650450736,
0.275726229,
-0.0043976414,
-0.1713227183,
-0.0783392489,
0.101006113,
0.1789459586,
0.5017950535,
-0.0036269187,
-0.1863487959,
-0.1349208206,
-0.06367746,
-0.0468236953,
0.0711337999,
-0.1824290454,
0.2553721368,
0.2577781379,
0.1839799583,
0.0547975227,
-0.1531580985,
-0.049743522,
0.0207578279,
0.2905255556,
-0.3741136193,
0.0461584181,
-0.1272042692,
0.0979504138,
-0.3777190149,
0.133810699,
-0.3502557576,
-0.1833579242,
-0.13573125,
-0.1108107418,
0.3093049228,
0.2101664692,
-0.3485353291,
0.1555946469,
0.2402639687,
-0.5443776846,
0.1576042026,
0.1009901762,
-0.3830035925,
0.0529526398,
0.1205425113,
-0.069621712,
-0.0969109163,
-0.1528278291,
0.0173261613,
-0.120101966,
-0.168003723,
0.1868709624,
-0.0529081486,
0.576834023,
-0.0123321833,
0.17038773,
-0.0075948229,
-0.0680121183,
0.1667571813,
-0.1843082756,
-0.1167619526,
-0.1657408476,
-0.1121703982,
0.0364884101,
-0.0776864365,
-0.622492671,
-0.4673991501,
-0.298817575,
-0.1805041134,
0.0007812897,
0.2649473846,
0.0936489254,
0.0102783879,
0.3407399356,
-0.0355759561,
0.0361260287,
-0.4035872817,
-0.2818410397,
0.2556054592,
0.0628467649,
-0.2947934866,
-0.0994649306,
0.0628204867,
0.2072018534,
-0.0956360623,
-0.3958401084,
-0.2739954591,
0.2307889462,
-0.0112172971,
-0.0910095274,
0.0472383387,
0.115157485,
-0.1613558531,
0.1061526015,
-0.2067127079,
-0.5061610341,
0.1450342834,
0.1470153183,
0.6205662489,
-0.1660098732,
0.2980053425,
-0.0526382141,
0.6775238514,
0.1890773475,
-0.22801736,
0.2107889056,
-0.2105232626,
0.3032868505,
0.0035383345,
-0.2769272923,
0.303943038,
0.0719058588,
0.0459163487,
0.3248113692,
0.0534217097,
-0.0166757554,
-0.0613192208,
0.3597290814,
-0.1392308474,
-0.1571272016,
-0.0605639182,
-0.0752927959,
-0.270031482,
0.0741318464,
0.3065281808,
-0.1772013009,
-0.167300418,
-0.1517760158,
0.4453921914,
-0.0859651268,
0.2577698827,
-0.6791189909,
-0.0043503684,
-0.1259898245,
0.2868065238,
-0.1449967921,
0.6176646352,
-0.0544952974,
-0.1857381314,
-0.0650188327,
0.0791010112,
0.4798837006,
-0.0459978692,
-0.0093407119,
-0.0361170769,
0.2946528494,
-0.6179637909,
-0.1878313869,
0.177603066,
0.168530792,
0.0936853215,
0.3528565466,
-0.1821607947,
-0.0771108866,
0.1613402218,
0.1991808563,
-0.1662609726,
-0.0170081481,
-0.3835246861,
-0.2073012292,
-0.3429888785,
-0.0511816852,
-0.2764179111,
0.1328060031,
0.1391487271,
0.1173347309,
0.2389437258,
-0.1128841564,
0.3738931715,
0.1367883533,
0.3835943937,
0.1973971426,
0.1353372186,
0.2334741801,
0.2617396414,
-0.1502474099,
0.4892303944,
-0.4564042687,
-0.300064683,
0.0343395285,
-0.0737036765,
0.1476983726,
0.3775241375,
-0.1436185092,
0.0837648883,
-0.3207883835,
-0.0862554684,
0.2754157186,
0.1629421562,
0.2510093153,
0.0771015659,
-0.1133953929,
-0.4098264277,
0.1798561662,
-0.1120343506,
-0.0426193736,
0.2145652324,
-0.4636404514,
-0.2888950408,
0.0964141116,
-0.1039727405,
0.8939821124,
-0.0649744123,
0.1889764816,
0.3377414048,
0.163262412,
0.282250911,
-0.0774448365,
0.1654772758,
-0.4589557946,
-0.0782303363,
0.0744743645,
-0.1392125636,
-0.0647865608,
0.3663624823,
0.0141664529,
0.5146530271,
-0.2688011825,
0.3116568029,
0.0540140532,
0.5210407972,
-0.1337995678,
-0.0108749662,
-0.3472366631,
0.1949187219,
-0.1809045076,
0.2802455127,
-0.120389536,
0.0054682009,
-0.3029420078,
-0.1379411668,
-0.1319408566,
0.2274258137,
-0.5376274586,
0.1669766158,
0.0934275612,
-0.348642379,
0.0191773418,
0.5643285513,
-0.1002913713,
-0.0820769295,
-0.1084555537,
0.3530824482,
0.0207635928,
0.3046308756,
0.0768878311,
0.045780655,
0.4243013263,
-0.0168561283,
-0.0948248357,
0.0720395148,
-0.0042468379,
-0.3094083369,
-0.2512998581,
0.0408150665,
0.3062187433,
-0.275687933,
-0.1972320974,
-0.1188385338,
0.0159462802,
-0.0596126914,
0.1198261082,
0.0611224659,
-0.0966570675,
0.2068723142,
0.1296120584,
-0.4074322283,
0.0401295908,
0.3414309621,
0.0581959039,
0.0030539334,
0.6670225859,
0.0741894022,
0.0704763979,
-0.1949027628,
0.1497740597,
0.3011733294,
-0.2336236238,
-0.0392005332,
-0.200078547,
-0.2490166873,
0.0004849647,
-0.1210952103,
0.2407470047,
-0.0176704507,
0.0402800739,
-0.4578700364,
-0.4342665076,
0.0315098315,
-0.0630611703,
0.0092654163,
0.0782027319,
-0.0439873487,
0.14506495,
0.0453661829,
-0.2561044991,
0.2107703835,
-0.1966145635,
0.2120199651,
0.0993525088,
-0.040149983,
0.2497250438,
-0.0228080787,
0.0503341965,
-0.0272153113,
-0.3081597984,
-0.1973446459,
-0.1295123994,
0.1182671338,
0.0220860038,
-0.0156389251,
-0.1738800704,
-0.2943679392,
-0.1543197334,
-0.2474768013,
-0.0346161574,
0.0881622881,
0.0274492465,
0.014234297,
-0.0534724034,
0.0265386216,
0.065787822,
-0.0739741847,
0.0375888459,
0.0440007299,
0.2452985197,
0.1012573615,
0.0479806922,
-0.0090397513,
-0.5060155988,
0.1013092324,
0.1878548414,
0.3835131526,
0.0299074557,
-0.1631865799,
-0.0384694189,
0.2729741931,
0.0583595075,
0.4147218466,
-0.4566238821,
-0.0442087837,
-0.0886463895,
0.1434324086,
-0.217178762,
0.0970661119,
0.3625799417,
-0.1833088547,
-0.0155576682,
0.2735647261,
0.1266647428,
0.1897180825,
0.0217409395,
0.106899038,
0.5871457458,
-0.0874020159,
0.1646407843,
0.2900646031,
-0.2160798907,
0.2205363363,
0.3349782526,
-0.0837545395,
-0.0087307738,
0.3994483054,
-0.1929350495,
0.3431118131,
-0.0790345296,
-0.1280988157,
0.2440440208,
-0.2488020658,
0.0799285248,
0.4024313986,
-0.1566055417,
0.1699330956,
0.046969384,
0.5395813584,
-0.4212686121,
-0.1374427974,
-0.0382388309,
-0.1659312993,
-0.1484350711,
-0.0598561019,
0.0648373216,
-0.1156119779,
-0.1001708359,
-0.2388542593,
-0.1484496295,
-0.3574168086,
-0.0503092706,
-0.0039663431,
-0.3478865921,
-0.0714052841,
-0.068698734,
0.3550386727,
-0.1964207739,
-0.1058575436,
0.3332425058,
-0.1416483521,
0.0370898917,
0.3267585635,
0.2570814192,
0.1953930259,
0.445263952,
0.0781016648,
-0.1183170602,
0.3155587018,
0.0447257794,
0.0146261593,
0.1478068382,
-0.1330284029,
-0.197568655,
0.3923157454,
0.1372192055,
-0.0548008978,
-0.2756022513,
0.3441490829,
-0.06653478,
-0.2472363561,
0.2151203603,
-0.2440630496,
-0.0380831659,
-0.5316841006,
0.3191307783,
-0.3130172789,
0.0980826393,
0.4218283296,
0.1543096006,
0.3799420595,
-0.2579368949,
0.0727035925,
-0.0288272742,
0.3897283077,
0.1729899645,
-0.2461687028,
-0.1826698333,
0.1881108582,
-0.9209600091,
0.4458394349,
0.0589725003,
-0.0390838832,
0.0334377028,
0.343989253,
0.053749647,
0.053144753,
0.2887403071,
-0.0962834731,
0.1624651998,
-0.0415592231,
-0.3789628446,
-0.3341311216,
-0.3106013238,
0.4049323201,
0.1494320631,
-0.5924780369,
0.2898758352,
0.0384889767,
-0.0501694456,
-0.1049866974,
-0.2132234871,
0.1590007693,
-0.1347790956,
0.4179120064,
-0.1402911395,
0.4938714206,
-0.0273788664,
-0.0191060323,
-0.2152628452,
-0.1111689806,
-0.295769453,
0.2690995634,
-0.1461154521,
0.0767934695,
0.1251029819,
0.0760324299,
-0.2987894714,
0.3234612346,
0.1469840556,
-0.2812111974,
-0.4066530764,
-0.021511659,
0.037754409,
0.0922570676,
0.0601234101,
0.3981384039,
-0.1498903483,
0.2334182113,
-0.1931377202,
-0.3373350799,
0.5052193403,
-0.3257140219,
-0.1926413476,
-0.274181962,
0.2520014048,
0.1326460689,
0.0052114832,
-0.6400470734,
0.0537734814,
0.3680358827,
-0.1895974576,
-0.1688361317,
0.1406752467,
-0.234420985,
0.4075177908,
0.0046435916,
0.0375307649,
0.0067872964,
-0.130524829,
0.1636308432,
-0.2550604045
]
|
https://github.com/huggingface/datasets/issues/1964 | Datasets.py function load_dataset does not match squad dataset | I'm glad you managed to fix run_qa.py for your case :)
Regarding the checksum error, I'm not able to reproduce on my side.
This errors says that the downloaded file doesn't match the expected file.
Could you try running this and let me know if you get the same output as me ?
```python
from datasets.utils.info_utils import get_size_checksum_dict
from datasets import cached_path
get_size_checksum_dict(cached_path("https://rajpurkar.github.io/SQuAD-explorer/dataset/train-v1.1.json"))
# {'num_bytes': 30288272, 'checksum': '3527663986b8295af4f7fcdff1ba1ff3f72d07d61a20f487cb238a6ef92fd955'}
``` | ### 1 When I try to train lxmert,and follow the code in README that --dataset name:
```shell
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --dataset_name squad --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
the bug is that:
```
Downloading and preparing dataset squad/plain_text (download: 33.51 MiB, generated: 85.75 MiB, post-processed: Unknown size, total: 119.27 MiB) to /home2/zhenggo1/.cache/huggingface/datasets/squad/plain_text/1.0.0/4c81550d83a2ac7c7ce23783bd8ff36642800e6633c1f18417fb58c3ff50cdd7...
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 217, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/load.py", line 746, in load_dataset
use_auth_token=use_auth_token,
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 573, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 633, in _download_and_prepare
self.info.download_checksums, dl_manager.get_recorded_sizes_checksums(), "dataset source files"
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/utils/info_utils.py", line 39, in verify_checksums
raise NonMatchingChecksumError(error_msg + str(bad_urls))
datasets.utils.info_utils.NonMatchingChecksumError: Checksums didn't match for dataset source files:
['https://rajpurkar.github.io/SQuAD-explorer/dataset/train-v1.1.json']
```
And I try to find the [checksum link](https://github.com/huggingface/datasets/blob/master/datasets/squad/dataset_infos.json)
,is the problem plain_text do not have a checksum?
### 2 When I try to train lxmert,and use local dataset:
```
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --train_file $SQUAD_DIR/train-v1.1.json --validation_file $SQUAD_DIR/dev-v1.1.json --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
The bug is that
```
['title', 'paragraphs']
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 273, in main
answer_column_name = "answers" if "answers" in column_names else column_names[2]
IndexError: list index out of range
```
I print the answer_column_name and find that local squad dataset need the package datasets to preprocessing so that the code below can work:
```
if training_args.do_train:
column_names = datasets["train"].column_names
else:
column_names = datasets["validation"].column_names
print(datasets["train"].column_names)
question_column_name = "question" if "question" in column_names else column_names[0]
context_column_name = "context" if "context" in column_names else column_names[1]
answer_column_name = "answers" if "answers" in column_names else column_names[2]
```
## Please tell me how to fix the bug,thks a lot! | 69 | Datasets.py function load_dataset does not match squad dataset
### 1 When I try to train lxmert,and follow the code in README that --dataset name:
```shell
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --dataset_name squad --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
the bug is that:
```
Downloading and preparing dataset squad/plain_text (download: 33.51 MiB, generated: 85.75 MiB, post-processed: Unknown size, total: 119.27 MiB) to /home2/zhenggo1/.cache/huggingface/datasets/squad/plain_text/1.0.0/4c81550d83a2ac7c7ce23783bd8ff36642800e6633c1f18417fb58c3ff50cdd7...
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 217, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/load.py", line 746, in load_dataset
use_auth_token=use_auth_token,
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 573, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 633, in _download_and_prepare
self.info.download_checksums, dl_manager.get_recorded_sizes_checksums(), "dataset source files"
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/utils/info_utils.py", line 39, in verify_checksums
raise NonMatchingChecksumError(error_msg + str(bad_urls))
datasets.utils.info_utils.NonMatchingChecksumError: Checksums didn't match for dataset source files:
['https://rajpurkar.github.io/SQuAD-explorer/dataset/train-v1.1.json']
```
And I try to find the [checksum link](https://github.com/huggingface/datasets/blob/master/datasets/squad/dataset_infos.json)
,is the problem plain_text do not have a checksum?
### 2 When I try to train lxmert,and use local dataset:
```
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --train_file $SQUAD_DIR/train-v1.1.json --validation_file $SQUAD_DIR/dev-v1.1.json --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
The bug is that
```
['title', 'paragraphs']
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 273, in main
answer_column_name = "answers" if "answers" in column_names else column_names[2]
IndexError: list index out of range
```
I print the answer_column_name and find that local squad dataset need the package datasets to preprocessing so that the code below can work:
```
if training_args.do_train:
column_names = datasets["train"].column_names
else:
column_names = datasets["validation"].column_names
print(datasets["train"].column_names)
question_column_name = "question" if "question" in column_names else column_names[0]
context_column_name = "context" if "context" in column_names else column_names[1]
answer_column_name = "answers" if "answers" in column_names else column_names[2]
```
## Please tell me how to fix the bug,thks a lot!
I'm glad you managed to fix run_qa.py for your case :)
Regarding the checksum error, I'm not able to reproduce on my side.
This errors says that the downloaded file doesn't match the expected file.
Could you try running this and let me know if you get the same output as me ?
```python
from datasets.utils.info_utils import get_size_checksum_dict
from datasets import cached_path
get_size_checksum_dict(cached_path("https://rajpurkar.github.io/SQuAD-explorer/dataset/train-v1.1.json"))
# {'num_bytes': 30288272, 'checksum': '3527663986b8295af4f7fcdff1ba1ff3f72d07d61a20f487cb238a6ef92fd955'}
``` | [
-0.353720516,
0.062508136,
0.0333653875,
0.3963990211,
0.5554528236,
0.0057210471,
0.5457585454,
0.3404690027,
-0.0983098298,
-0.1216232702,
-0.1650660038,
0.4508371055,
0.1084151044,
-0.0774194002,
0.1859293282,
0.2271615863,
-0.1242240593,
-0.0123368576,
-0.146108225,
-0.2893434763,
-0.1109782234,
0.1282913834,
-0.0823142305,
0.2603986561,
-0.1788989604,
0.0166908335,
0.2006343305,
0.272364527,
-0.0037961234,
-0.2199689448,
0.3428422213,
-0.2606053054,
0.2361017913,
0.5758854747,
-0.0001148581,
0.1132559404,
-0.0115429182,
-0.3397287428,
-0.5327041745,
-0.200222224,
-0.1021838337,
-0.3757724166,
0.1486450732,
-0.2605550587,
-0.1595322788,
0.0937372819,
0.0203168094,
-0.4645365775,
0.3544548452,
0.4883139133,
0.2139826268,
0.2522563934,
-0.1001007184,
0.0775510296,
0.1142543554,
-0.0009760716,
-0.0695089027,
0.1360990107,
0.334751159,
-0.0582687482,
0.0025544665,
0.1724458039,
0.0828082114,
0.0568235703,
0.2703093886,
0.00640395,
0.1991152614,
-0.316155225,
0.0564420745,
0.3177836835,
0.4627766013,
-0.4630154967,
-0.3347049356,
-0.2631345689,
0.1331043243,
-0.2565730214,
0.132016629,
0.0672779083,
0.0511588715,
-0.0424275659,
0.0477761962,
0.0376647562,
0.1114289686,
0.0368288346,
0.0068917884,
0.1999943256,
0.0288671236,
0.2383964211,
-0.2015398741,
-0.113548398,
0.1148922071,
-0.0831490755,
0.081831947,
0.3924934268,
-0.8285178542,
-0.0814113691,
-0.204295367,
-0.1421722323,
0.2562874258,
0.0819008574,
0.0386919901,
-0.0269713923,
0.1486689448,
0.1044572815,
0.1687799692,
0.3692039847,
0.3052024841,
0.2674018741,
-0.0663637966,
0.0668205172,
-0.3717198074,
0.2254105806,
-0.3225814104,
-0.1618057787,
0.0960094184,
0.0835883096,
0.0062301527,
-0.1927383393,
-0.5517168641,
0.1761803031,
0.1544087827,
0.0026421717,
0.097437188,
0.3247915506,
0.0759161711,
0.3802294135,
0.0263225865,
0.10485553,
-0.2946235538,
-0.3704166412,
-0.2408784926,
0.0330557562,
-0.088010259,
0.0268362667,
0.0183389839,
-0.0356005244,
0.3227836788,
-0.1957619935,
0.2114949375,
-0.1790798306,
0.1150043458,
-0.2556710541,
-0.1285900921,
-0.0065956297,
0.029810125,
0.0322049223,
0.1742972434,
-0.3730716109,
-0.0482526384,
0.1222927868,
-0.3712682724,
-0.033652354,
-0.1820592135,
0.187116161,
-0.1391082406,
0.0208787471,
-0.2373691797,
0.1698869169,
0.3073554337,
-0.1878398061,
0.1063958183,
-0.3961227238,
-0.1664003581,
-0.2122270465,
0.3151643872,
0.3882878423,
-0.407456696,
-0.2117101997,
0.1249394417,
-0.0952139422,
-0.0381637625,
0.1216654554,
-0.1516054869,
0.1555612236,
-0.2062239051,
-0.0070254449,
0.7145841718,
-0.8443274498,
-0.4243375659,
0.1099731028,
-0.3059237003,
-0.0194986593,
-0.0710132122,
0.0664077923,
0.1930086017,
0.0147336926,
0.0827302188,
0.5066138506,
-0.0362929925,
0.0914033353,
-0.1724579334,
-0.2712132037,
0.2526646852,
0.1708514988,
0.0318142623,
-0.0380644612,
0.0388375632,
0.4989835918,
0.332213819,
0.1426165998,
0.1112047285,
-0.0299586449,
-0.035939917,
-0.0821635276,
0.1146057397,
-0.1481087208,
-0.6798555851,
0.163865611,
-0.1644513905,
0.0439886115,
0.241078347,
-0.1508303434,
-0.2135134488,
-0.0917366818,
-0.4252308309,
-0.1023876891,
0.0743928775,
0.1495984048,
-0.0585199855,
-0.1226448193,
-0.1598846763,
0.3514792919,
-0.2154511809,
0.2284578085,
-0.4849060178,
-0.0965390131,
-0.0637454763,
-0.0362155698,
0.0537011735,
0.3318409622,
0.0871920362,
-0.0709578618,
-0.1057533845,
0.4979955852,
0.0180258043,
0.1023962423,
0.2883630097,
-0.2016446739,
0.0996332839,
-0.0990449861,
0.0020645163,
0.2259094566,
0.1946659386,
-0.0812614039,
0.0224433318,
0.1418917328,
0.1574392319,
0.0746555328,
-0.004860986,
-0.1536110938,
0.1390525997,
0.0118647087,
0.0111015849,
0.0971625224,
0.0520382561,
0.2940090001,
0.5483118296,
0.1111360267,
-0.1868931949,
0.1310920715,
0.3315557837,
-0.0650450736,
0.275726229,
-0.0043976414,
-0.1713227183,
-0.0783392489,
0.101006113,
0.1789459586,
0.5017950535,
-0.0036269187,
-0.1863487959,
-0.1349208206,
-0.06367746,
-0.0468236953,
0.0711337999,
-0.1824290454,
0.2553721368,
0.2577781379,
0.1839799583,
0.0547975227,
-0.1531580985,
-0.049743522,
0.0207578279,
0.2905255556,
-0.3741136193,
0.0461584181,
-0.1272042692,
0.0979504138,
-0.3777190149,
0.133810699,
-0.3502557576,
-0.1833579242,
-0.13573125,
-0.1108107418,
0.3093049228,
0.2101664692,
-0.3485353291,
0.1555946469,
0.2402639687,
-0.5443776846,
0.1576042026,
0.1009901762,
-0.3830035925,
0.0529526398,
0.1205425113,
-0.069621712,
-0.0969109163,
-0.1528278291,
0.0173261613,
-0.120101966,
-0.168003723,
0.1868709624,
-0.0529081486,
0.576834023,
-0.0123321833,
0.17038773,
-0.0075948229,
-0.0680121183,
0.1667571813,
-0.1843082756,
-0.1167619526,
-0.1657408476,
-0.1121703982,
0.0364884101,
-0.0776864365,
-0.622492671,
-0.4673991501,
-0.298817575,
-0.1805041134,
0.0007812897,
0.2649473846,
0.0936489254,
0.0102783879,
0.3407399356,
-0.0355759561,
0.0361260287,
-0.4035872817,
-0.2818410397,
0.2556054592,
0.0628467649,
-0.2947934866,
-0.0994649306,
0.0628204867,
0.2072018534,
-0.0956360623,
-0.3958401084,
-0.2739954591,
0.2307889462,
-0.0112172971,
-0.0910095274,
0.0472383387,
0.115157485,
-0.1613558531,
0.1061526015,
-0.2067127079,
-0.5061610341,
0.1450342834,
0.1470153183,
0.6205662489,
-0.1660098732,
0.2980053425,
-0.0526382141,
0.6775238514,
0.1890773475,
-0.22801736,
0.2107889056,
-0.2105232626,
0.3032868505,
0.0035383345,
-0.2769272923,
0.303943038,
0.0719058588,
0.0459163487,
0.3248113692,
0.0534217097,
-0.0166757554,
-0.0613192208,
0.3597290814,
-0.1392308474,
-0.1571272016,
-0.0605639182,
-0.0752927959,
-0.270031482,
0.0741318464,
0.3065281808,
-0.1772013009,
-0.167300418,
-0.1517760158,
0.4453921914,
-0.0859651268,
0.2577698827,
-0.6791189909,
-0.0043503684,
-0.1259898245,
0.2868065238,
-0.1449967921,
0.6176646352,
-0.0544952974,
-0.1857381314,
-0.0650188327,
0.0791010112,
0.4798837006,
-0.0459978692,
-0.0093407119,
-0.0361170769,
0.2946528494,
-0.6179637909,
-0.1878313869,
0.177603066,
0.168530792,
0.0936853215,
0.3528565466,
-0.1821607947,
-0.0771108866,
0.1613402218,
0.1991808563,
-0.1662609726,
-0.0170081481,
-0.3835246861,
-0.2073012292,
-0.3429888785,
-0.0511816852,
-0.2764179111,
0.1328060031,
0.1391487271,
0.1173347309,
0.2389437258,
-0.1128841564,
0.3738931715,
0.1367883533,
0.3835943937,
0.1973971426,
0.1353372186,
0.2334741801,
0.2617396414,
-0.1502474099,
0.4892303944,
-0.4564042687,
-0.300064683,
0.0343395285,
-0.0737036765,
0.1476983726,
0.3775241375,
-0.1436185092,
0.0837648883,
-0.3207883835,
-0.0862554684,
0.2754157186,
0.1629421562,
0.2510093153,
0.0771015659,
-0.1133953929,
-0.4098264277,
0.1798561662,
-0.1120343506,
-0.0426193736,
0.2145652324,
-0.4636404514,
-0.2888950408,
0.0964141116,
-0.1039727405,
0.8939821124,
-0.0649744123,
0.1889764816,
0.3377414048,
0.163262412,
0.282250911,
-0.0774448365,
0.1654772758,
-0.4589557946,
-0.0782303363,
0.0744743645,
-0.1392125636,
-0.0647865608,
0.3663624823,
0.0141664529,
0.5146530271,
-0.2688011825,
0.3116568029,
0.0540140532,
0.5210407972,
-0.1337995678,
-0.0108749662,
-0.3472366631,
0.1949187219,
-0.1809045076,
0.2802455127,
-0.120389536,
0.0054682009,
-0.3029420078,
-0.1379411668,
-0.1319408566,
0.2274258137,
-0.5376274586,
0.1669766158,
0.0934275612,
-0.348642379,
0.0191773418,
0.5643285513,
-0.1002913713,
-0.0820769295,
-0.1084555537,
0.3530824482,
0.0207635928,
0.3046308756,
0.0768878311,
0.045780655,
0.4243013263,
-0.0168561283,
-0.0948248357,
0.0720395148,
-0.0042468379,
-0.3094083369,
-0.2512998581,
0.0408150665,
0.3062187433,
-0.275687933,
-0.1972320974,
-0.1188385338,
0.0159462802,
-0.0596126914,
0.1198261082,
0.0611224659,
-0.0966570675,
0.2068723142,
0.1296120584,
-0.4074322283,
0.0401295908,
0.3414309621,
0.0581959039,
0.0030539334,
0.6670225859,
0.0741894022,
0.0704763979,
-0.1949027628,
0.1497740597,
0.3011733294,
-0.2336236238,
-0.0392005332,
-0.200078547,
-0.2490166873,
0.0004849647,
-0.1210952103,
0.2407470047,
-0.0176704507,
0.0402800739,
-0.4578700364,
-0.4342665076,
0.0315098315,
-0.0630611703,
0.0092654163,
0.0782027319,
-0.0439873487,
0.14506495,
0.0453661829,
-0.2561044991,
0.2107703835,
-0.1966145635,
0.2120199651,
0.0993525088,
-0.040149983,
0.2497250438,
-0.0228080787,
0.0503341965,
-0.0272153113,
-0.3081597984,
-0.1973446459,
-0.1295123994,
0.1182671338,
0.0220860038,
-0.0156389251,
-0.1738800704,
-0.2943679392,
-0.1543197334,
-0.2474768013,
-0.0346161574,
0.0881622881,
0.0274492465,
0.014234297,
-0.0534724034,
0.0265386216,
0.065787822,
-0.0739741847,
0.0375888459,
0.0440007299,
0.2452985197,
0.1012573615,
0.0479806922,
-0.0090397513,
-0.5060155988,
0.1013092324,
0.1878548414,
0.3835131526,
0.0299074557,
-0.1631865799,
-0.0384694189,
0.2729741931,
0.0583595075,
0.4147218466,
-0.4566238821,
-0.0442087837,
-0.0886463895,
0.1434324086,
-0.217178762,
0.0970661119,
0.3625799417,
-0.1833088547,
-0.0155576682,
0.2735647261,
0.1266647428,
0.1897180825,
0.0217409395,
0.106899038,
0.5871457458,
-0.0874020159,
0.1646407843,
0.2900646031,
-0.2160798907,
0.2205363363,
0.3349782526,
-0.0837545395,
-0.0087307738,
0.3994483054,
-0.1929350495,
0.3431118131,
-0.0790345296,
-0.1280988157,
0.2440440208,
-0.2488020658,
0.0799285248,
0.4024313986,
-0.1566055417,
0.1699330956,
0.046969384,
0.5395813584,
-0.4212686121,
-0.1374427974,
-0.0382388309,
-0.1659312993,
-0.1484350711,
-0.0598561019,
0.0648373216,
-0.1156119779,
-0.1001708359,
-0.2388542593,
-0.1484496295,
-0.3574168086,
-0.0503092706,
-0.0039663431,
-0.3478865921,
-0.0714052841,
-0.068698734,
0.3550386727,
-0.1964207739,
-0.1058575436,
0.3332425058,
-0.1416483521,
0.0370898917,
0.3267585635,
0.2570814192,
0.1953930259,
0.445263952,
0.0781016648,
-0.1183170602,
0.3155587018,
0.0447257794,
0.0146261593,
0.1478068382,
-0.1330284029,
-0.197568655,
0.3923157454,
0.1372192055,
-0.0548008978,
-0.2756022513,
0.3441490829,
-0.06653478,
-0.2472363561,
0.2151203603,
-0.2440630496,
-0.0380831659,
-0.5316841006,
0.3191307783,
-0.3130172789,
0.0980826393,
0.4218283296,
0.1543096006,
0.3799420595,
-0.2579368949,
0.0727035925,
-0.0288272742,
0.3897283077,
0.1729899645,
-0.2461687028,
-0.1826698333,
0.1881108582,
-0.9209600091,
0.4458394349,
0.0589725003,
-0.0390838832,
0.0334377028,
0.343989253,
0.053749647,
0.053144753,
0.2887403071,
-0.0962834731,
0.1624651998,
-0.0415592231,
-0.3789628446,
-0.3341311216,
-0.3106013238,
0.4049323201,
0.1494320631,
-0.5924780369,
0.2898758352,
0.0384889767,
-0.0501694456,
-0.1049866974,
-0.2132234871,
0.1590007693,
-0.1347790956,
0.4179120064,
-0.1402911395,
0.4938714206,
-0.0273788664,
-0.0191060323,
-0.2152628452,
-0.1111689806,
-0.295769453,
0.2690995634,
-0.1461154521,
0.0767934695,
0.1251029819,
0.0760324299,
-0.2987894714,
0.3234612346,
0.1469840556,
-0.2812111974,
-0.4066530764,
-0.021511659,
0.037754409,
0.0922570676,
0.0601234101,
0.3981384039,
-0.1498903483,
0.2334182113,
-0.1931377202,
-0.3373350799,
0.5052193403,
-0.3257140219,
-0.1926413476,
-0.274181962,
0.2520014048,
0.1326460689,
0.0052114832,
-0.6400470734,
0.0537734814,
0.3680358827,
-0.1895974576,
-0.1688361317,
0.1406752467,
-0.234420985,
0.4075177908,
0.0046435916,
0.0375307649,
0.0067872964,
-0.130524829,
0.1636308432,
-0.2550604045
]
|
https://github.com/huggingface/datasets/issues/1964 | Datasets.py function load_dataset does not match squad dataset | I run the code,and it show below:
```
>>> from datasets.utils.info_utils import get_size_checksum_dict
>>> from datasets import cached_path
>>> get_size_checksum_dict(cached_path("https://rajpurkar.github.io/SQuAD-explorer/dataset/train-v1.1.json"))
Downloading: 30.3MB [04:13, 120kB/s]
{'num_bytes': 30288272, 'checksum': '3527663986b8295af4f7fcdff1ba1ff3f72d07d61a20f487cb238a6ef92fd955'}
``` | ### 1 When I try to train lxmert,and follow the code in README that --dataset name:
```shell
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --dataset_name squad --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
the bug is that:
```
Downloading and preparing dataset squad/plain_text (download: 33.51 MiB, generated: 85.75 MiB, post-processed: Unknown size, total: 119.27 MiB) to /home2/zhenggo1/.cache/huggingface/datasets/squad/plain_text/1.0.0/4c81550d83a2ac7c7ce23783bd8ff36642800e6633c1f18417fb58c3ff50cdd7...
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 217, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/load.py", line 746, in load_dataset
use_auth_token=use_auth_token,
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 573, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 633, in _download_and_prepare
self.info.download_checksums, dl_manager.get_recorded_sizes_checksums(), "dataset source files"
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/utils/info_utils.py", line 39, in verify_checksums
raise NonMatchingChecksumError(error_msg + str(bad_urls))
datasets.utils.info_utils.NonMatchingChecksumError: Checksums didn't match for dataset source files:
['https://rajpurkar.github.io/SQuAD-explorer/dataset/train-v1.1.json']
```
And I try to find the [checksum link](https://github.com/huggingface/datasets/blob/master/datasets/squad/dataset_infos.json)
,is the problem plain_text do not have a checksum?
### 2 When I try to train lxmert,and use local dataset:
```
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --train_file $SQUAD_DIR/train-v1.1.json --validation_file $SQUAD_DIR/dev-v1.1.json --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
The bug is that
```
['title', 'paragraphs']
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 273, in main
answer_column_name = "answers" if "answers" in column_names else column_names[2]
IndexError: list index out of range
```
I print the answer_column_name and find that local squad dataset need the package datasets to preprocessing so that the code below can work:
```
if training_args.do_train:
column_names = datasets["train"].column_names
else:
column_names = datasets["validation"].column_names
print(datasets["train"].column_names)
question_column_name = "question" if "question" in column_names else column_names[0]
context_column_name = "context" if "context" in column_names else column_names[1]
answer_column_name = "answers" if "answers" in column_names else column_names[2]
```
## Please tell me how to fix the bug,thks a lot! | 29 | Datasets.py function load_dataset does not match squad dataset
### 1 When I try to train lxmert,and follow the code in README that --dataset name:
```shell
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --dataset_name squad --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
the bug is that:
```
Downloading and preparing dataset squad/plain_text (download: 33.51 MiB, generated: 85.75 MiB, post-processed: Unknown size, total: 119.27 MiB) to /home2/zhenggo1/.cache/huggingface/datasets/squad/plain_text/1.0.0/4c81550d83a2ac7c7ce23783bd8ff36642800e6633c1f18417fb58c3ff50cdd7...
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 217, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/load.py", line 746, in load_dataset
use_auth_token=use_auth_token,
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 573, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 633, in _download_and_prepare
self.info.download_checksums, dl_manager.get_recorded_sizes_checksums(), "dataset source files"
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/utils/info_utils.py", line 39, in verify_checksums
raise NonMatchingChecksumError(error_msg + str(bad_urls))
datasets.utils.info_utils.NonMatchingChecksumError: Checksums didn't match for dataset source files:
['https://rajpurkar.github.io/SQuAD-explorer/dataset/train-v1.1.json']
```
And I try to find the [checksum link](https://github.com/huggingface/datasets/blob/master/datasets/squad/dataset_infos.json)
,is the problem plain_text do not have a checksum?
### 2 When I try to train lxmert,and use local dataset:
```
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --train_file $SQUAD_DIR/train-v1.1.json --validation_file $SQUAD_DIR/dev-v1.1.json --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
The bug is that
```
['title', 'paragraphs']
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 273, in main
answer_column_name = "answers" if "answers" in column_names else column_names[2]
IndexError: list index out of range
```
I print the answer_column_name and find that local squad dataset need the package datasets to preprocessing so that the code below can work:
```
if training_args.do_train:
column_names = datasets["train"].column_names
else:
column_names = datasets["validation"].column_names
print(datasets["train"].column_names)
question_column_name = "question" if "question" in column_names else column_names[0]
context_column_name = "context" if "context" in column_names else column_names[1]
answer_column_name = "answers" if "answers" in column_names else column_names[2]
```
## Please tell me how to fix the bug,thks a lot!
I run the code,and it show below:
```
>>> from datasets.utils.info_utils import get_size_checksum_dict
>>> from datasets import cached_path
>>> get_size_checksum_dict(cached_path("https://rajpurkar.github.io/SQuAD-explorer/dataset/train-v1.1.json"))
Downloading: 30.3MB [04:13, 120kB/s]
{'num_bytes': 30288272, 'checksum': '3527663986b8295af4f7fcdff1ba1ff3f72d07d61a20f487cb238a6ef92fd955'}
``` | [
-0.353720516,
0.062508136,
0.0333653875,
0.3963990211,
0.5554528236,
0.0057210471,
0.5457585454,
0.3404690027,
-0.0983098298,
-0.1216232702,
-0.1650660038,
0.4508371055,
0.1084151044,
-0.0774194002,
0.1859293282,
0.2271615863,
-0.1242240593,
-0.0123368576,
-0.146108225,
-0.2893434763,
-0.1109782234,
0.1282913834,
-0.0823142305,
0.2603986561,
-0.1788989604,
0.0166908335,
0.2006343305,
0.272364527,
-0.0037961234,
-0.2199689448,
0.3428422213,
-0.2606053054,
0.2361017913,
0.5758854747,
-0.0001148581,
0.1132559404,
-0.0115429182,
-0.3397287428,
-0.5327041745,
-0.200222224,
-0.1021838337,
-0.3757724166,
0.1486450732,
-0.2605550587,
-0.1595322788,
0.0937372819,
0.0203168094,
-0.4645365775,
0.3544548452,
0.4883139133,
0.2139826268,
0.2522563934,
-0.1001007184,
0.0775510296,
0.1142543554,
-0.0009760716,
-0.0695089027,
0.1360990107,
0.334751159,
-0.0582687482,
0.0025544665,
0.1724458039,
0.0828082114,
0.0568235703,
0.2703093886,
0.00640395,
0.1991152614,
-0.316155225,
0.0564420745,
0.3177836835,
0.4627766013,
-0.4630154967,
-0.3347049356,
-0.2631345689,
0.1331043243,
-0.2565730214,
0.132016629,
0.0672779083,
0.0511588715,
-0.0424275659,
0.0477761962,
0.0376647562,
0.1114289686,
0.0368288346,
0.0068917884,
0.1999943256,
0.0288671236,
0.2383964211,
-0.2015398741,
-0.113548398,
0.1148922071,
-0.0831490755,
0.081831947,
0.3924934268,
-0.8285178542,
-0.0814113691,
-0.204295367,
-0.1421722323,
0.2562874258,
0.0819008574,
0.0386919901,
-0.0269713923,
0.1486689448,
0.1044572815,
0.1687799692,
0.3692039847,
0.3052024841,
0.2674018741,
-0.0663637966,
0.0668205172,
-0.3717198074,
0.2254105806,
-0.3225814104,
-0.1618057787,
0.0960094184,
0.0835883096,
0.0062301527,
-0.1927383393,
-0.5517168641,
0.1761803031,
0.1544087827,
0.0026421717,
0.097437188,
0.3247915506,
0.0759161711,
0.3802294135,
0.0263225865,
0.10485553,
-0.2946235538,
-0.3704166412,
-0.2408784926,
0.0330557562,
-0.088010259,
0.0268362667,
0.0183389839,
-0.0356005244,
0.3227836788,
-0.1957619935,
0.2114949375,
-0.1790798306,
0.1150043458,
-0.2556710541,
-0.1285900921,
-0.0065956297,
0.029810125,
0.0322049223,
0.1742972434,
-0.3730716109,
-0.0482526384,
0.1222927868,
-0.3712682724,
-0.033652354,
-0.1820592135,
0.187116161,
-0.1391082406,
0.0208787471,
-0.2373691797,
0.1698869169,
0.3073554337,
-0.1878398061,
0.1063958183,
-0.3961227238,
-0.1664003581,
-0.2122270465,
0.3151643872,
0.3882878423,
-0.407456696,
-0.2117101997,
0.1249394417,
-0.0952139422,
-0.0381637625,
0.1216654554,
-0.1516054869,
0.1555612236,
-0.2062239051,
-0.0070254449,
0.7145841718,
-0.8443274498,
-0.4243375659,
0.1099731028,
-0.3059237003,
-0.0194986593,
-0.0710132122,
0.0664077923,
0.1930086017,
0.0147336926,
0.0827302188,
0.5066138506,
-0.0362929925,
0.0914033353,
-0.1724579334,
-0.2712132037,
0.2526646852,
0.1708514988,
0.0318142623,
-0.0380644612,
0.0388375632,
0.4989835918,
0.332213819,
0.1426165998,
0.1112047285,
-0.0299586449,
-0.035939917,
-0.0821635276,
0.1146057397,
-0.1481087208,
-0.6798555851,
0.163865611,
-0.1644513905,
0.0439886115,
0.241078347,
-0.1508303434,
-0.2135134488,
-0.0917366818,
-0.4252308309,
-0.1023876891,
0.0743928775,
0.1495984048,
-0.0585199855,
-0.1226448193,
-0.1598846763,
0.3514792919,
-0.2154511809,
0.2284578085,
-0.4849060178,
-0.0965390131,
-0.0637454763,
-0.0362155698,
0.0537011735,
0.3318409622,
0.0871920362,
-0.0709578618,
-0.1057533845,
0.4979955852,
0.0180258043,
0.1023962423,
0.2883630097,
-0.2016446739,
0.0996332839,
-0.0990449861,
0.0020645163,
0.2259094566,
0.1946659386,
-0.0812614039,
0.0224433318,
0.1418917328,
0.1574392319,
0.0746555328,
-0.004860986,
-0.1536110938,
0.1390525997,
0.0118647087,
0.0111015849,
0.0971625224,
0.0520382561,
0.2940090001,
0.5483118296,
0.1111360267,
-0.1868931949,
0.1310920715,
0.3315557837,
-0.0650450736,
0.275726229,
-0.0043976414,
-0.1713227183,
-0.0783392489,
0.101006113,
0.1789459586,
0.5017950535,
-0.0036269187,
-0.1863487959,
-0.1349208206,
-0.06367746,
-0.0468236953,
0.0711337999,
-0.1824290454,
0.2553721368,
0.2577781379,
0.1839799583,
0.0547975227,
-0.1531580985,
-0.049743522,
0.0207578279,
0.2905255556,
-0.3741136193,
0.0461584181,
-0.1272042692,
0.0979504138,
-0.3777190149,
0.133810699,
-0.3502557576,
-0.1833579242,
-0.13573125,
-0.1108107418,
0.3093049228,
0.2101664692,
-0.3485353291,
0.1555946469,
0.2402639687,
-0.5443776846,
0.1576042026,
0.1009901762,
-0.3830035925,
0.0529526398,
0.1205425113,
-0.069621712,
-0.0969109163,
-0.1528278291,
0.0173261613,
-0.120101966,
-0.168003723,
0.1868709624,
-0.0529081486,
0.576834023,
-0.0123321833,
0.17038773,
-0.0075948229,
-0.0680121183,
0.1667571813,
-0.1843082756,
-0.1167619526,
-0.1657408476,
-0.1121703982,
0.0364884101,
-0.0776864365,
-0.622492671,
-0.4673991501,
-0.298817575,
-0.1805041134,
0.0007812897,
0.2649473846,
0.0936489254,
0.0102783879,
0.3407399356,
-0.0355759561,
0.0361260287,
-0.4035872817,
-0.2818410397,
0.2556054592,
0.0628467649,
-0.2947934866,
-0.0994649306,
0.0628204867,
0.2072018534,
-0.0956360623,
-0.3958401084,
-0.2739954591,
0.2307889462,
-0.0112172971,
-0.0910095274,
0.0472383387,
0.115157485,
-0.1613558531,
0.1061526015,
-0.2067127079,
-0.5061610341,
0.1450342834,
0.1470153183,
0.6205662489,
-0.1660098732,
0.2980053425,
-0.0526382141,
0.6775238514,
0.1890773475,
-0.22801736,
0.2107889056,
-0.2105232626,
0.3032868505,
0.0035383345,
-0.2769272923,
0.303943038,
0.0719058588,
0.0459163487,
0.3248113692,
0.0534217097,
-0.0166757554,
-0.0613192208,
0.3597290814,
-0.1392308474,
-0.1571272016,
-0.0605639182,
-0.0752927959,
-0.270031482,
0.0741318464,
0.3065281808,
-0.1772013009,
-0.167300418,
-0.1517760158,
0.4453921914,
-0.0859651268,
0.2577698827,
-0.6791189909,
-0.0043503684,
-0.1259898245,
0.2868065238,
-0.1449967921,
0.6176646352,
-0.0544952974,
-0.1857381314,
-0.0650188327,
0.0791010112,
0.4798837006,
-0.0459978692,
-0.0093407119,
-0.0361170769,
0.2946528494,
-0.6179637909,
-0.1878313869,
0.177603066,
0.168530792,
0.0936853215,
0.3528565466,
-0.1821607947,
-0.0771108866,
0.1613402218,
0.1991808563,
-0.1662609726,
-0.0170081481,
-0.3835246861,
-0.2073012292,
-0.3429888785,
-0.0511816852,
-0.2764179111,
0.1328060031,
0.1391487271,
0.1173347309,
0.2389437258,
-0.1128841564,
0.3738931715,
0.1367883533,
0.3835943937,
0.1973971426,
0.1353372186,
0.2334741801,
0.2617396414,
-0.1502474099,
0.4892303944,
-0.4564042687,
-0.300064683,
0.0343395285,
-0.0737036765,
0.1476983726,
0.3775241375,
-0.1436185092,
0.0837648883,
-0.3207883835,
-0.0862554684,
0.2754157186,
0.1629421562,
0.2510093153,
0.0771015659,
-0.1133953929,
-0.4098264277,
0.1798561662,
-0.1120343506,
-0.0426193736,
0.2145652324,
-0.4636404514,
-0.2888950408,
0.0964141116,
-0.1039727405,
0.8939821124,
-0.0649744123,
0.1889764816,
0.3377414048,
0.163262412,
0.282250911,
-0.0774448365,
0.1654772758,
-0.4589557946,
-0.0782303363,
0.0744743645,
-0.1392125636,
-0.0647865608,
0.3663624823,
0.0141664529,
0.5146530271,
-0.2688011825,
0.3116568029,
0.0540140532,
0.5210407972,
-0.1337995678,
-0.0108749662,
-0.3472366631,
0.1949187219,
-0.1809045076,
0.2802455127,
-0.120389536,
0.0054682009,
-0.3029420078,
-0.1379411668,
-0.1319408566,
0.2274258137,
-0.5376274586,
0.1669766158,
0.0934275612,
-0.348642379,
0.0191773418,
0.5643285513,
-0.1002913713,
-0.0820769295,
-0.1084555537,
0.3530824482,
0.0207635928,
0.3046308756,
0.0768878311,
0.045780655,
0.4243013263,
-0.0168561283,
-0.0948248357,
0.0720395148,
-0.0042468379,
-0.3094083369,
-0.2512998581,
0.0408150665,
0.3062187433,
-0.275687933,
-0.1972320974,
-0.1188385338,
0.0159462802,
-0.0596126914,
0.1198261082,
0.0611224659,
-0.0966570675,
0.2068723142,
0.1296120584,
-0.4074322283,
0.0401295908,
0.3414309621,
0.0581959039,
0.0030539334,
0.6670225859,
0.0741894022,
0.0704763979,
-0.1949027628,
0.1497740597,
0.3011733294,
-0.2336236238,
-0.0392005332,
-0.200078547,
-0.2490166873,
0.0004849647,
-0.1210952103,
0.2407470047,
-0.0176704507,
0.0402800739,
-0.4578700364,
-0.4342665076,
0.0315098315,
-0.0630611703,
0.0092654163,
0.0782027319,
-0.0439873487,
0.14506495,
0.0453661829,
-0.2561044991,
0.2107703835,
-0.1966145635,
0.2120199651,
0.0993525088,
-0.040149983,
0.2497250438,
-0.0228080787,
0.0503341965,
-0.0272153113,
-0.3081597984,
-0.1973446459,
-0.1295123994,
0.1182671338,
0.0220860038,
-0.0156389251,
-0.1738800704,
-0.2943679392,
-0.1543197334,
-0.2474768013,
-0.0346161574,
0.0881622881,
0.0274492465,
0.014234297,
-0.0534724034,
0.0265386216,
0.065787822,
-0.0739741847,
0.0375888459,
0.0440007299,
0.2452985197,
0.1012573615,
0.0479806922,
-0.0090397513,
-0.5060155988,
0.1013092324,
0.1878548414,
0.3835131526,
0.0299074557,
-0.1631865799,
-0.0384694189,
0.2729741931,
0.0583595075,
0.4147218466,
-0.4566238821,
-0.0442087837,
-0.0886463895,
0.1434324086,
-0.217178762,
0.0970661119,
0.3625799417,
-0.1833088547,
-0.0155576682,
0.2735647261,
0.1266647428,
0.1897180825,
0.0217409395,
0.106899038,
0.5871457458,
-0.0874020159,
0.1646407843,
0.2900646031,
-0.2160798907,
0.2205363363,
0.3349782526,
-0.0837545395,
-0.0087307738,
0.3994483054,
-0.1929350495,
0.3431118131,
-0.0790345296,
-0.1280988157,
0.2440440208,
-0.2488020658,
0.0799285248,
0.4024313986,
-0.1566055417,
0.1699330956,
0.046969384,
0.5395813584,
-0.4212686121,
-0.1374427974,
-0.0382388309,
-0.1659312993,
-0.1484350711,
-0.0598561019,
0.0648373216,
-0.1156119779,
-0.1001708359,
-0.2388542593,
-0.1484496295,
-0.3574168086,
-0.0503092706,
-0.0039663431,
-0.3478865921,
-0.0714052841,
-0.068698734,
0.3550386727,
-0.1964207739,
-0.1058575436,
0.3332425058,
-0.1416483521,
0.0370898917,
0.3267585635,
0.2570814192,
0.1953930259,
0.445263952,
0.0781016648,
-0.1183170602,
0.3155587018,
0.0447257794,
0.0146261593,
0.1478068382,
-0.1330284029,
-0.197568655,
0.3923157454,
0.1372192055,
-0.0548008978,
-0.2756022513,
0.3441490829,
-0.06653478,
-0.2472363561,
0.2151203603,
-0.2440630496,
-0.0380831659,
-0.5316841006,
0.3191307783,
-0.3130172789,
0.0980826393,
0.4218283296,
0.1543096006,
0.3799420595,
-0.2579368949,
0.0727035925,
-0.0288272742,
0.3897283077,
0.1729899645,
-0.2461687028,
-0.1826698333,
0.1881108582,
-0.9209600091,
0.4458394349,
0.0589725003,
-0.0390838832,
0.0334377028,
0.343989253,
0.053749647,
0.053144753,
0.2887403071,
-0.0962834731,
0.1624651998,
-0.0415592231,
-0.3789628446,
-0.3341311216,
-0.3106013238,
0.4049323201,
0.1494320631,
-0.5924780369,
0.2898758352,
0.0384889767,
-0.0501694456,
-0.1049866974,
-0.2132234871,
0.1590007693,
-0.1347790956,
0.4179120064,
-0.1402911395,
0.4938714206,
-0.0273788664,
-0.0191060323,
-0.2152628452,
-0.1111689806,
-0.295769453,
0.2690995634,
-0.1461154521,
0.0767934695,
0.1251029819,
0.0760324299,
-0.2987894714,
0.3234612346,
0.1469840556,
-0.2812111974,
-0.4066530764,
-0.021511659,
0.037754409,
0.0922570676,
0.0601234101,
0.3981384039,
-0.1498903483,
0.2334182113,
-0.1931377202,
-0.3373350799,
0.5052193403,
-0.3257140219,
-0.1926413476,
-0.274181962,
0.2520014048,
0.1326460689,
0.0052114832,
-0.6400470734,
0.0537734814,
0.3680358827,
-0.1895974576,
-0.1688361317,
0.1406752467,
-0.234420985,
0.4075177908,
0.0046435916,
0.0375307649,
0.0067872964,
-0.130524829,
0.1636308432,
-0.2550604045
]
|
https://github.com/huggingface/datasets/issues/1964 | Datasets.py function load_dataset does not match squad dataset | Alright ! So in this case redownloading the file with `download_mode="force_redownload"` should fix it. Can you try using `download_mode="force_redownload"` again ?
Not sure why it didn't work for you the first time though :/ | ### 1 When I try to train lxmert,and follow the code in README that --dataset name:
```shell
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --dataset_name squad --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
the bug is that:
```
Downloading and preparing dataset squad/plain_text (download: 33.51 MiB, generated: 85.75 MiB, post-processed: Unknown size, total: 119.27 MiB) to /home2/zhenggo1/.cache/huggingface/datasets/squad/plain_text/1.0.0/4c81550d83a2ac7c7ce23783bd8ff36642800e6633c1f18417fb58c3ff50cdd7...
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 217, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/load.py", line 746, in load_dataset
use_auth_token=use_auth_token,
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 573, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 633, in _download_and_prepare
self.info.download_checksums, dl_manager.get_recorded_sizes_checksums(), "dataset source files"
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/utils/info_utils.py", line 39, in verify_checksums
raise NonMatchingChecksumError(error_msg + str(bad_urls))
datasets.utils.info_utils.NonMatchingChecksumError: Checksums didn't match for dataset source files:
['https://rajpurkar.github.io/SQuAD-explorer/dataset/train-v1.1.json']
```
And I try to find the [checksum link](https://github.com/huggingface/datasets/blob/master/datasets/squad/dataset_infos.json)
,is the problem plain_text do not have a checksum?
### 2 When I try to train lxmert,and use local dataset:
```
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --train_file $SQUAD_DIR/train-v1.1.json --validation_file $SQUAD_DIR/dev-v1.1.json --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
The bug is that
```
['title', 'paragraphs']
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 273, in main
answer_column_name = "answers" if "answers" in column_names else column_names[2]
IndexError: list index out of range
```
I print the answer_column_name and find that local squad dataset need the package datasets to preprocessing so that the code below can work:
```
if training_args.do_train:
column_names = datasets["train"].column_names
else:
column_names = datasets["validation"].column_names
print(datasets["train"].column_names)
question_column_name = "question" if "question" in column_names else column_names[0]
context_column_name = "context" if "context" in column_names else column_names[1]
answer_column_name = "answers" if "answers" in column_names else column_names[2]
```
## Please tell me how to fix the bug,thks a lot! | 34 | Datasets.py function load_dataset does not match squad dataset
### 1 When I try to train lxmert,and follow the code in README that --dataset name:
```shell
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --dataset_name squad --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
the bug is that:
```
Downloading and preparing dataset squad/plain_text (download: 33.51 MiB, generated: 85.75 MiB, post-processed: Unknown size, total: 119.27 MiB) to /home2/zhenggo1/.cache/huggingface/datasets/squad/plain_text/1.0.0/4c81550d83a2ac7c7ce23783bd8ff36642800e6633c1f18417fb58c3ff50cdd7...
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 217, in main
datasets = load_dataset(data_args.dataset_name, data_args.dataset_config_name)
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/load.py", line 746, in load_dataset
use_auth_token=use_auth_token,
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 573, in download_and_prepare
dl_manager=dl_manager, verify_infos=verify_infos, **download_and_prepare_kwargs
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/builder.py", line 633, in _download_and_prepare
self.info.download_checksums, dl_manager.get_recorded_sizes_checksums(), "dataset source files"
File "/home2/zhenggo1/anaconda3/envs/lpot/lib/python3.7/site-packages/datasets/utils/info_utils.py", line 39, in verify_checksums
raise NonMatchingChecksumError(error_msg + str(bad_urls))
datasets.utils.info_utils.NonMatchingChecksumError: Checksums didn't match for dataset source files:
['https://rajpurkar.github.io/SQuAD-explorer/dataset/train-v1.1.json']
```
And I try to find the [checksum link](https://github.com/huggingface/datasets/blob/master/datasets/squad/dataset_infos.json)
,is the problem plain_text do not have a checksum?
### 2 When I try to train lxmert,and use local dataset:
```
python examples/question-answering/run_qa.py --model_name_or_path unc-nlp/lxmert-base-uncased --train_file $SQUAD_DIR/train-v1.1.json --validation_file $SQUAD_DIR/dev-v1.1.json --do_train --do_eval --per_device_train_batch_size 12 --learning_rate 3e-5 --num_train_epochs 2 --max_seq_length 384 --doc_stride 128 --output_dir /home2/zhenggo1/checkpoint/lxmert_squad
```
The bug is that
```
['title', 'paragraphs']
Traceback (most recent call last):
File "examples/question-answering/run_qa.py", line 501, in <module>
main()
File "examples/question-answering/run_qa.py", line 273, in main
answer_column_name = "answers" if "answers" in column_names else column_names[2]
IndexError: list index out of range
```
I print the answer_column_name and find that local squad dataset need the package datasets to preprocessing so that the code below can work:
```
if training_args.do_train:
column_names = datasets["train"].column_names
else:
column_names = datasets["validation"].column_names
print(datasets["train"].column_names)
question_column_name = "question" if "question" in column_names else column_names[0]
context_column_name = "context" if "context" in column_names else column_names[1]
answer_column_name = "answers" if "answers" in column_names else column_names[2]
```
## Please tell me how to fix the bug,thks a lot!
Alright ! So in this case redownloading the file with `download_mode="force_redownload"` should fix it. Can you try using `download_mode="force_redownload"` again ?
Not sure why it didn't work for you the first time though :/ | [
-0.353720516,
0.062508136,
0.0333653875,
0.3963990211,
0.5554528236,
0.0057210471,
0.5457585454,
0.3404690027,
-0.0983098298,
-0.1216232702,
-0.1650660038,
0.4508371055,
0.1084151044,
-0.0774194002,
0.1859293282,
0.2271615863,
-0.1242240593,
-0.0123368576,
-0.146108225,
-0.2893434763,
-0.1109782234,
0.1282913834,
-0.0823142305,
0.2603986561,
-0.1788989604,
0.0166908335,
0.2006343305,
0.272364527,
-0.0037961234,
-0.2199689448,
0.3428422213,
-0.2606053054,
0.2361017913,
0.5758854747,
-0.0001148581,
0.1132559404,
-0.0115429182,
-0.3397287428,
-0.5327041745,
-0.200222224,
-0.1021838337,
-0.3757724166,
0.1486450732,
-0.2605550587,
-0.1595322788,
0.0937372819,
0.0203168094,
-0.4645365775,
0.3544548452,
0.4883139133,
0.2139826268,
0.2522563934,
-0.1001007184,
0.0775510296,
0.1142543554,
-0.0009760716,
-0.0695089027,
0.1360990107,
0.334751159,
-0.0582687482,
0.0025544665,
0.1724458039,
0.0828082114,
0.0568235703,
0.2703093886,
0.00640395,
0.1991152614,
-0.316155225,
0.0564420745,
0.3177836835,
0.4627766013,
-0.4630154967,
-0.3347049356,
-0.2631345689,
0.1331043243,
-0.2565730214,
0.132016629,
0.0672779083,
0.0511588715,
-0.0424275659,
0.0477761962,
0.0376647562,
0.1114289686,
0.0368288346,
0.0068917884,
0.1999943256,
0.0288671236,
0.2383964211,
-0.2015398741,
-0.113548398,
0.1148922071,
-0.0831490755,
0.081831947,
0.3924934268,
-0.8285178542,
-0.0814113691,
-0.204295367,
-0.1421722323,
0.2562874258,
0.0819008574,
0.0386919901,
-0.0269713923,
0.1486689448,
0.1044572815,
0.1687799692,
0.3692039847,
0.3052024841,
0.2674018741,
-0.0663637966,
0.0668205172,
-0.3717198074,
0.2254105806,
-0.3225814104,
-0.1618057787,
0.0960094184,
0.0835883096,
0.0062301527,
-0.1927383393,
-0.5517168641,
0.1761803031,
0.1544087827,
0.0026421717,
0.097437188,
0.3247915506,
0.0759161711,
0.3802294135,
0.0263225865,
0.10485553,
-0.2946235538,
-0.3704166412,
-0.2408784926,
0.0330557562,
-0.088010259,
0.0268362667,
0.0183389839,
-0.0356005244,
0.3227836788,
-0.1957619935,
0.2114949375,
-0.1790798306,
0.1150043458,
-0.2556710541,
-0.1285900921,
-0.0065956297,
0.029810125,
0.0322049223,
0.1742972434,
-0.3730716109,
-0.0482526384,
0.1222927868,
-0.3712682724,
-0.033652354,
-0.1820592135,
0.187116161,
-0.1391082406,
0.0208787471,
-0.2373691797,
0.1698869169,
0.3073554337,
-0.1878398061,
0.1063958183,
-0.3961227238,
-0.1664003581,
-0.2122270465,
0.3151643872,
0.3882878423,
-0.407456696,
-0.2117101997,
0.1249394417,
-0.0952139422,
-0.0381637625,
0.1216654554,
-0.1516054869,
0.1555612236,
-0.2062239051,
-0.0070254449,
0.7145841718,
-0.8443274498,
-0.4243375659,
0.1099731028,
-0.3059237003,
-0.0194986593,
-0.0710132122,
0.0664077923,
0.1930086017,
0.0147336926,
0.0827302188,
0.5066138506,
-0.0362929925,
0.0914033353,
-0.1724579334,
-0.2712132037,
0.2526646852,
0.1708514988,
0.0318142623,
-0.0380644612,
0.0388375632,
0.4989835918,
0.332213819,
0.1426165998,
0.1112047285,
-0.0299586449,
-0.035939917,
-0.0821635276,
0.1146057397,
-0.1481087208,
-0.6798555851,
0.163865611,
-0.1644513905,
0.0439886115,
0.241078347,
-0.1508303434,
-0.2135134488,
-0.0917366818,
-0.4252308309,
-0.1023876891,
0.0743928775,
0.1495984048,
-0.0585199855,
-0.1226448193,
-0.1598846763,
0.3514792919,
-0.2154511809,
0.2284578085,
-0.4849060178,
-0.0965390131,
-0.0637454763,
-0.0362155698,
0.0537011735,
0.3318409622,
0.0871920362,
-0.0709578618,
-0.1057533845,
0.4979955852,
0.0180258043,
0.1023962423,
0.2883630097,
-0.2016446739,
0.0996332839,
-0.0990449861,
0.0020645163,
0.2259094566,
0.1946659386,
-0.0812614039,
0.0224433318,
0.1418917328,
0.1574392319,
0.0746555328,
-0.004860986,
-0.1536110938,
0.1390525997,
0.0118647087,
0.0111015849,
0.0971625224,
0.0520382561,
0.2940090001,
0.5483118296,
0.1111360267,
-0.1868931949,
0.1310920715,
0.3315557837,
-0.0650450736,
0.275726229,
-0.0043976414,
-0.1713227183,
-0.0783392489,
0.101006113,
0.1789459586,
0.5017950535,
-0.0036269187,
-0.1863487959,
-0.1349208206,
-0.06367746,
-0.0468236953,
0.0711337999,
-0.1824290454,
0.2553721368,
0.2577781379,
0.1839799583,
0.0547975227,
-0.1531580985,
-0.049743522,
0.0207578279,
0.2905255556,
-0.3741136193,
0.0461584181,
-0.1272042692,
0.0979504138,
-0.3777190149,
0.133810699,
-0.3502557576,
-0.1833579242,
-0.13573125,
-0.1108107418,
0.3093049228,
0.2101664692,
-0.3485353291,
0.1555946469,
0.2402639687,
-0.5443776846,
0.1576042026,
0.1009901762,
-0.3830035925,
0.0529526398,
0.1205425113,
-0.069621712,
-0.0969109163,
-0.1528278291,
0.0173261613,
-0.120101966,
-0.168003723,
0.1868709624,
-0.0529081486,
0.576834023,
-0.0123321833,
0.17038773,
-0.0075948229,
-0.0680121183,
0.1667571813,
-0.1843082756,
-0.1167619526,
-0.1657408476,
-0.1121703982,
0.0364884101,
-0.0776864365,
-0.622492671,
-0.4673991501,
-0.298817575,
-0.1805041134,
0.0007812897,
0.2649473846,
0.0936489254,
0.0102783879,
0.3407399356,
-0.0355759561,
0.0361260287,
-0.4035872817,
-0.2818410397,
0.2556054592,
0.0628467649,
-0.2947934866,
-0.0994649306,
0.0628204867,
0.2072018534,
-0.0956360623,
-0.3958401084,
-0.2739954591,
0.2307889462,
-0.0112172971,
-0.0910095274,
0.0472383387,
0.115157485,
-0.1613558531,
0.1061526015,
-0.2067127079,
-0.5061610341,
0.1450342834,
0.1470153183,
0.6205662489,
-0.1660098732,
0.2980053425,
-0.0526382141,
0.6775238514,
0.1890773475,
-0.22801736,
0.2107889056,
-0.2105232626,
0.3032868505,
0.0035383345,
-0.2769272923,
0.303943038,
0.0719058588,
0.0459163487,
0.3248113692,
0.0534217097,
-0.0166757554,
-0.0613192208,
0.3597290814,
-0.1392308474,
-0.1571272016,
-0.0605639182,
-0.0752927959,
-0.270031482,
0.0741318464,
0.3065281808,
-0.1772013009,
-0.167300418,
-0.1517760158,
0.4453921914,
-0.0859651268,
0.2577698827,
-0.6791189909,
-0.0043503684,
-0.1259898245,
0.2868065238,
-0.1449967921,
0.6176646352,
-0.0544952974,
-0.1857381314,
-0.0650188327,
0.0791010112,
0.4798837006,
-0.0459978692,
-0.0093407119,
-0.0361170769,
0.2946528494,
-0.6179637909,
-0.1878313869,
0.177603066,
0.168530792,
0.0936853215,
0.3528565466,
-0.1821607947,
-0.0771108866,
0.1613402218,
0.1991808563,
-0.1662609726,
-0.0170081481,
-0.3835246861,
-0.2073012292,
-0.3429888785,
-0.0511816852,
-0.2764179111,
0.1328060031,
0.1391487271,
0.1173347309,
0.2389437258,
-0.1128841564,
0.3738931715,
0.1367883533,
0.3835943937,
0.1973971426,
0.1353372186,
0.2334741801,
0.2617396414,
-0.1502474099,
0.4892303944,
-0.4564042687,
-0.300064683,
0.0343395285,
-0.0737036765,
0.1476983726,
0.3775241375,
-0.1436185092,
0.0837648883,
-0.3207883835,
-0.0862554684,
0.2754157186,
0.1629421562,
0.2510093153,
0.0771015659,
-0.1133953929,
-0.4098264277,
0.1798561662,
-0.1120343506,
-0.0426193736,
0.2145652324,
-0.4636404514,
-0.2888950408,
0.0964141116,
-0.1039727405,
0.8939821124,
-0.0649744123,
0.1889764816,
0.3377414048,
0.163262412,
0.282250911,
-0.0774448365,
0.1654772758,
-0.4589557946,
-0.0782303363,
0.0744743645,
-0.1392125636,
-0.0647865608,
0.3663624823,
0.0141664529,
0.5146530271,
-0.2688011825,
0.3116568029,
0.0540140532,
0.5210407972,
-0.1337995678,
-0.0108749662,
-0.3472366631,
0.1949187219,
-0.1809045076,
0.2802455127,
-0.120389536,
0.0054682009,
-0.3029420078,
-0.1379411668,
-0.1319408566,
0.2274258137,
-0.5376274586,
0.1669766158,
0.0934275612,
-0.348642379,
0.0191773418,
0.5643285513,
-0.1002913713,
-0.0820769295,
-0.1084555537,
0.3530824482,
0.0207635928,
0.3046308756,
0.0768878311,
0.045780655,
0.4243013263,
-0.0168561283,
-0.0948248357,
0.0720395148,
-0.0042468379,
-0.3094083369,
-0.2512998581,
0.0408150665,
0.3062187433,
-0.275687933,
-0.1972320974,
-0.1188385338,
0.0159462802,
-0.0596126914,
0.1198261082,
0.0611224659,
-0.0966570675,
0.2068723142,
0.1296120584,
-0.4074322283,
0.0401295908,
0.3414309621,
0.0581959039,
0.0030539334,
0.6670225859,
0.0741894022,
0.0704763979,
-0.1949027628,
0.1497740597,
0.3011733294,
-0.2336236238,
-0.0392005332,
-0.200078547,
-0.2490166873,
0.0004849647,
-0.1210952103,
0.2407470047,
-0.0176704507,
0.0402800739,
-0.4578700364,
-0.4342665076,
0.0315098315,
-0.0630611703,
0.0092654163,
0.0782027319,
-0.0439873487,
0.14506495,
0.0453661829,
-0.2561044991,
0.2107703835,
-0.1966145635,
0.2120199651,
0.0993525088,
-0.040149983,
0.2497250438,
-0.0228080787,
0.0503341965,
-0.0272153113,
-0.3081597984,
-0.1973446459,
-0.1295123994,
0.1182671338,
0.0220860038,
-0.0156389251,
-0.1738800704,
-0.2943679392,
-0.1543197334,
-0.2474768013,
-0.0346161574,
0.0881622881,
0.0274492465,
0.014234297,
-0.0534724034,
0.0265386216,
0.065787822,
-0.0739741847,
0.0375888459,
0.0440007299,
0.2452985197,
0.1012573615,
0.0479806922,
-0.0090397513,
-0.5060155988,
0.1013092324,
0.1878548414,
0.3835131526,
0.0299074557,
-0.1631865799,
-0.0384694189,
0.2729741931,
0.0583595075,
0.4147218466,
-0.4566238821,
-0.0442087837,
-0.0886463895,
0.1434324086,
-0.217178762,
0.0970661119,
0.3625799417,
-0.1833088547,
-0.0155576682,
0.2735647261,
0.1266647428,
0.1897180825,
0.0217409395,
0.106899038,
0.5871457458,
-0.0874020159,
0.1646407843,
0.2900646031,
-0.2160798907,
0.2205363363,
0.3349782526,
-0.0837545395,
-0.0087307738,
0.3994483054,
-0.1929350495,
0.3431118131,
-0.0790345296,
-0.1280988157,
0.2440440208,
-0.2488020658,
0.0799285248,
0.4024313986,
-0.1566055417,
0.1699330956,
0.046969384,
0.5395813584,
-0.4212686121,
-0.1374427974,
-0.0382388309,
-0.1659312993,
-0.1484350711,
-0.0598561019,
0.0648373216,
-0.1156119779,
-0.1001708359,
-0.2388542593,
-0.1484496295,
-0.3574168086,
-0.0503092706,
-0.0039663431,
-0.3478865921,
-0.0714052841,
-0.068698734,
0.3550386727,
-0.1964207739,
-0.1058575436,
0.3332425058,
-0.1416483521,
0.0370898917,
0.3267585635,
0.2570814192,
0.1953930259,
0.445263952,
0.0781016648,
-0.1183170602,
0.3155587018,
0.0447257794,
0.0146261593,
0.1478068382,
-0.1330284029,
-0.197568655,
0.3923157454,
0.1372192055,
-0.0548008978,
-0.2756022513,
0.3441490829,
-0.06653478,
-0.2472363561,
0.2151203603,
-0.2440630496,
-0.0380831659,
-0.5316841006,
0.3191307783,
-0.3130172789,
0.0980826393,
0.4218283296,
0.1543096006,
0.3799420595,
-0.2579368949,
0.0727035925,
-0.0288272742,
0.3897283077,
0.1729899645,
-0.2461687028,
-0.1826698333,
0.1881108582,
-0.9209600091,
0.4458394349,
0.0589725003,
-0.0390838832,
0.0334377028,
0.343989253,
0.053749647,
0.053144753,
0.2887403071,
-0.0962834731,
0.1624651998,
-0.0415592231,
-0.3789628446,
-0.3341311216,
-0.3106013238,
0.4049323201,
0.1494320631,
-0.5924780369,
0.2898758352,
0.0384889767,
-0.0501694456,
-0.1049866974,
-0.2132234871,
0.1590007693,
-0.1347790956,
0.4179120064,
-0.1402911395,
0.4938714206,
-0.0273788664,
-0.0191060323,
-0.2152628452,
-0.1111689806,
-0.295769453,
0.2690995634,
-0.1461154521,
0.0767934695,
0.1251029819,
0.0760324299,
-0.2987894714,
0.3234612346,
0.1469840556,
-0.2812111974,
-0.4066530764,
-0.021511659,
0.037754409,
0.0922570676,
0.0601234101,
0.3981384039,
-0.1498903483,
0.2334182113,
-0.1931377202,
-0.3373350799,
0.5052193403,
-0.3257140219,
-0.1926413476,
-0.274181962,
0.2520014048,
0.1326460689,
0.0052114832,
-0.6400470734,
0.0537734814,
0.3680358827,
-0.1895974576,
-0.1688361317,
0.1406752467,
-0.234420985,
0.4075177908,
0.0046435916,
0.0375307649,
0.0067872964,
-0.130524829,
0.1636308432,
-0.2550604045
]
|
https://github.com/huggingface/datasets/issues/1963 | bug in SNLI dataset | Hi ! The labels -1 correspond to the examples without gold labels in the original snli dataset.
Feel free to remove these examples if you don't need them by using
```python
data = data.filter(lambda x: x["label"] != -1)
``` | Hi
There is label of -1 in train set of SNLI dataset, please find the code below:
```
import numpy as np
import datasets
data = datasets.load_dataset("snli")["train"]
labels = []
for d in data:
labels.append(d["label"])
print(np.unique(labels))
```
and results:
`[-1 0 1 2]`
version of datasets used:
`datasets 1.2.1 <pip>
`
thanks for your help. @lhoestq | 39 | bug in SNLI dataset
Hi
There is label of -1 in train set of SNLI dataset, please find the code below:
```
import numpy as np
import datasets
data = datasets.load_dataset("snli")["train"]
labels = []
for d in data:
labels.append(d["label"])
print(np.unique(labels))
```
and results:
`[-1 0 1 2]`
version of datasets used:
`datasets 1.2.1 <pip>
`
thanks for your help. @lhoestq
Hi ! The labels -1 correspond to the examples without gold labels in the original snli dataset.
Feel free to remove these examples if you don't need them by using
```python
data = data.filter(lambda x: x["label"] != -1)
``` | [
0.1758221835,
-0.2825256586,
-0.1035732925,
0.3496331871,
0.2219780236,
0.0471981317,
0.3874209523,
0.118343696,
0.0808188692,
0.2969246209,
-0.2027284056,
0.6121096611,
-0.1427201033,
0.0958904251,
0.0018212005,
-0.0009764511,
0.2330547422,
0.3379024863,
0.2097957134,
-0.4966839254,
-0.2359666079,
-0.0235045366,
-0.3817799985,
0.265283078,
-0.2869724333,
0.1784488857,
-0.0155610554,
-0.1333380193,
0.1334051639,
-0.4898707271,
0.1604640633,
-0.0986121371,
0.1226141453,
0.2325981557,
-0.0001058666,
-0.2743191719,
0.1129735187,
0.0678980947,
-0.3696537316,
-0.2605842948,
-0.1101467013,
-0.0220088996,
-0.1468922198,
-0.1628938615,
-0.2096336186,
-0.1724424809,
0.014252089,
0.0935407504,
0.1124736145,
0.2662245929,
0.2374287993,
0.067387864,
-0.1264113188,
0.1347338706,
0.2391383052,
-0.3994210064,
0.1405960023,
0.113914445,
0.0496283919,
0.0493755937,
0.405608505,
0.6512392759,
-0.0620474704,
-0.043695543,
0.113712579,
0.0903739631,
0.127295047,
-0.3254042566,
-0.0787116513,
0.3404749632,
0.109191902,
-0.3263962865,
-0.4188061953,
0.0663316324,
0.1710608006,
-0.462331295,
-0.0283235162,
0.2538722754,
0.1406978369,
0.2034576535,
0.0178657416,
0.0447487198,
-0.0742186904,
0.3183242083,
0.0500646345,
0.6590716839,
-0.0445929728,
0.1819167286,
0.0840543434,
0.0472338907,
-0.1453343183,
0.1597238779,
0.0685572624,
0.3448962271,
-0.5432955027,
-0.0704534575,
-0.0477734767,
-0.0830214098,
-0.1924339086,
0.1565317959,
0.1354234517,
0.0860434622,
-0.1599018723,
0.2858230472,
-0.0184927527,
0.0122399321,
0.1436229646,
0.3150082529,
0.1404027343,
-0.2455684394,
0.0978090763,
-0.0502134934,
-0.1937008202,
-0.2610457838,
0.4902791679,
0.0590682812,
0.0314711519,
-0.0605651662,
-0.3164854646,
0.2388176471,
-0.3513686657,
0.0303708222,
-0.0477259271,
0.0027171802,
-0.0162866265,
-0.0127465026,
-0.0026859601,
0.0518940873,
0.0969412923,
-0.2098994255,
-0.265170306,
0.0638556629,
-0.0640471876,
-0.151625514,
-0.0483410135,
-0.138133958,
0.1749139279,
0.0200464074,
-0.3853111863,
-0.1688892692,
0.1255016178,
0.0578713715,
0.2358459979,
0.176627025,
-0.2485657483,
0.4699510932,
-0.1297380477,
-0.146986261,
0.043061655,
-0.006361431,
-0.2545924783,
-0.1627763957,
-0.3255552948,
0.2545197904,
0.0457577594,
-0.03966932,
0.0741636604,
-0.1403100491,
0.1460748315,
0.0239899214,
0.3011032343,
-0.2547023594,
-0.1741401851,
0.0351589285,
0.1350890696,
-0.0714571476,
-0.1860976666,
-0.1540897042,
-0.149802953,
-0.2205897272,
0.2482094169,
0.3248289227,
0.0830717832,
-0.0982794836,
-0.1811050624,
0.1455696076,
0.6714298129,
-0.4713219404,
-0.5759912133,
-0.037369974,
-0.3127925694,
-0.3483293056,
-0.0722914562,
0.5257781744,
-0.2541718483,
0.1841239631,
0.0559160635,
-0.0284528993,
0.0702486038,
-0.040790692,
-0.4820292294,
-0.2443046421,
0.4282409847,
0.287972033,
0.1184490025,
0.0484844856,
-0.0653522089,
-0.1245332807,
0.3911314607,
0.0004526134,
0.1557037532,
0.2652797401,
0.5162930489,
0.1337871253,
0.1086845621,
-0.2567135394,
-0.0195434019,
0.1996148229,
-0.1025180519,
0.3829931021,
0.1140017956,
-0.0327499658,
-0.2263216078,
-0.2294125259,
-0.371419847,
-0.1633772105,
0.1727943271,
0.1106387526,
0.12496005,
-0.0002136691,
-0.0690634325,
0.2349173427,
-0.1951017976,
0.0574190617,
-0.2534842789,
0.1734798998,
-0.0722109005,
0.0002442254,
-0.2396927923,
0.1996299922,
0.1941969991,
0.1150441319,
-0.1352174282,
0.2393589318,
-0.286143899,
-0.2572815418,
0.1280990988,
0.0865209401,
0.0745256394,
-0.6160662174,
-0.2318004966,
0.5943589211,
0.1214365736,
0.0328818262,
-0.1761131734,
0.205939129,
-0.1515799165,
-0.1561627686,
-0.3645667434,
0.2804862261,
0.1399027556,
-0.0466481894,
-0.0844029784,
-0.3972606361,
-0.0248351917,
-0.492898494,
0.3556154072,
0.1288474053,
-0.3839580417,
-0.0716296211,
0.3216439784,
-0.0507560112,
0.1607384086,
-0.0180259161,
-0.1524935365,
0.0302999467,
0.2578043938,
0.1180636957,
0.1480194032,
0.1922577769,
-0.0845909864,
0.1927809268,
-0.152892977,
-0.1079918891,
-0.0311147626,
0.1146150604,
-0.0190104526,
0.0687716305,
0.2076685727,
0.1067494974,
-0.1778415889,
-0.2461327314,
-0.1395559311,
0.2681661248,
-0.2324053198,
-0.0760472193,
-0.1674966514,
-0.2094909251,
-0.5057199001,
-0.1521826237,
-0.050710775,
-0.0992072374,
0.1847669333,
-0.0889074504,
-0.0105203269,
0.3142929077,
0.1960568577,
0.101229161,
0.1451550573,
0.1357939094,
-0.1855858564,
-0.3651708961,
-0.2351709455,
0.0717459023,
-0.3185688555,
0.2699111998,
0.3629637063,
-0.1586461067,
-0.0961318165,
-0.0891949236,
-0.244194746,
-0.0196913946,
0.0663823411,
0.0541801229,
0.0247740988,
-0.0128801046,
-0.2053204477,
0.2964855134,
0.2392023951,
0.0107820164,
-0.0804007277,
0.1651143283,
-0.0892628357,
-0.0484053418,
-0.2911716402,
-0.4643014967,
-0.5519580245,
0.0023524484,
0.0160964504,
-0.17084831,
0.1917138994,
0.1217432991,
0.0539651811,
-0.0223813299,
0.151152119,
0.1787068099,
-0.2152537107,
-0.1564624757,
0.1699819565,
0.0465794839,
-0.2690292001,
-0.1365803778,
0.0395993926,
-0.0324099511,
-0.1843264252,
-0.256113857,
-0.1648023874,
0.1473015845,
0.107036002,
0.0568780079,
0.0316864438,
-0.0339901522,
0.1185098067,
-0.0793801919,
-0.2076564431,
0.1215782166,
0.1340608448,
-0.3273539543,
0.4176498353,
-0.025411861,
0.297583729,
-0.0089342408,
0.0571755841,
0.6455110908,
-0.1563931257,
0.025855083,
-0.1597404331,
0.0220551677,
0.1431917995,
-0.2573869228,
0.1051396877,
0.4043575227,
-0.0375114232,
0.1361237019,
-0.0309335031,
0.0562434494,
0.0022190821,
0.1778100729,
-0.2866261899,
-0.0421883166,
-0.2883039713,
0.0081805103,
0.0121035157,
0.0024793723,
-0.129776746,
-0.0169941988,
-0.1806281954,
0.0000505573,
0.128452003,
-0.1970236897,
0.1025350913,
-0.2461903691,
-0.1150919348,
0.0435950048,
-0.1480238289,
0.1687986553,
0.3643044829,
0.1497537643,
0.16440323,
0.2874141932,
0.2821747065,
0.6061438322,
-0.5048745275,
0.2027049214,
0.2420894802,
0.4977799952,
-0.3383360803,
-0.3108196259,
-0.4152241647,
-0.2385451794,
0.4774546623,
0.1917116344,
0.0423534177,
-0.1758729964,
0.2739938796,
0.1112618297,
-0.3431811631,
-0.1644868851,
-0.2301605791,
-0.0410896353,
-0.1127400398,
0.1340053082,
-0.0636912286,
0.1891909838,
-0.0973165333,
-0.2146482766,
0.0549490191,
0.1077018902,
0.2498281747,
0.1666043997,
0.1703023165,
0.1368240118,
0.088375628,
0.0453658439,
0.124310486,
0.0230898168,
0.3792884052,
-0.150814563,
-0.3487549424,
-0.0490252413,
-0.4373478293,
0.0748114064,
-0.0211909004,
-0.0076100412,
-0.0620713048,
-0.1066274047,
0.3135819733,
0.0335743278,
0.1250332147,
0.0776630044,
0.2430591881,
-0.400236398,
-0.3817458153,
0.5641040802,
0.1614265591,
-0.3126894832,
0.1298294365,
-0.1115403399,
-0.232385844,
0.2495945245,
0.1741066277,
0.8710391521,
-0.043997217,
-0.0777831078,
0.187261641,
-0.5491983294,
0.4160414338,
-0.0962840319,
-0.156722784,
-0.5003465414,
0.0170136727,
0.0462397002,
0.0295671504,
-0.3262170255,
0.3848619759,
-0.0168425571,
0.3335964084,
-0.0721830577,
0.1315604001,
0.0626659989,
-0.0263761859,
0.3919154406,
0.1101384982,
-0.1359795928,
0.1998817623,
-0.0867550597,
0.365097791,
-0.1807791442,
0.0051468429,
-0.1593273729,
0.0511811599,
-0.1213786975,
-0.0190218166,
0.1898372173,
0.2132985592,
0.0453389324,
-0.1771476865,
-0.337167263,
0.3609566092,
0.7153921723,
0.0166355465,
-0.003223517,
0.0695010126,
0.2405068576,
0.4848551154,
0.0438016206,
0.2695523202,
0.4295511842,
-0.0175232757,
-0.3549792767,
0.1668362021,
0.1424151808,
0.1523371041,
-0.4034621716,
0.0467119478,
-0.1067344546,
0.184780851,
0.1023439914,
-0.0712310523,
0.2050367594,
-0.2408464104,
0.1963704675,
0.0711249709,
-0.1680080295,
0.0077843349,
-0.0060905055,
-0.2926675677,
-0.1471734643,
0.3525080681,
0.1104489937,
0.1597244292,
-0.0106635252,
-0.1213549078,
-0.0746301636,
-0.2623015344,
-0.0025097025,
0.2081126869,
-0.4890864789,
0.1244214401,
-0.0861644298,
0.0198645033,
-0.1201538444,
0.2211391628,
0.0576065965,
0.2330546826,
0.1238742694,
-0.293453902,
-0.4125736952,
-0.1974737346,
0.0958213359,
0.3416712284,
-0.1268660575,
0.1598139405,
-0.1841472089,
-0.1776507795,
-0.3772062659,
-0.051179681,
-0.2954213917,
0.2258237302,
0.1912424415,
0.2830271721,
-0.1852221638,
0.0047181076,
0.1705357581,
-0.0288294144,
-0.0857435241,
-0.2370089293,
-0.2462337017,
0.0528249964,
0.2717521787,
-0.2514607012,
-0.0944942906,
0.1243267357,
0.0607837513,
0.0364014469,
0.2265709043,
0.247709915,
0.2358562052,
0.9074829221,
-0.1937107742,
0.0112096658,
-0.0809666812,
-0.0974370316,
0.0123712178,
0.0009786543,
0.0554629937,
0.0699673668,
0.1669415981,
0.0968657285,
-0.2715978324,
-0.1088640317,
-0.3038879931,
-0.0061019077,
0.010286889,
-0.5755169392,
0.4176028073,
0.2746882737,
0.2566926777,
0.0816469267,
-0.3946270943,
-0.2472676635,
0.2157204747,
0.3618087471,
-0.2296126038,
-0.006482318,
0.2608965039,
0.1352832317,
0.0575640351,
0.0501226149,
-0.0629425868,
-0.1292919815,
-0.0882651508,
0.0369224139,
0.1134366766,
-0.3506599963,
0.0868881568,
0.4115596414,
0.0686953142,
0.0167494621,
0.1330555379,
-0.084811233,
-0.1213137656,
0.6410638094,
-0.0264557656,
0.4369126558,
-0.0552829243,
0.1763094068,
-0.231853947,
0.1728788018,
-0.063804701,
0.1332532614,
-0.4932236671,
0.0759487301,
-0.0145824449,
0.2183900326,
-0.0881679803,
-0.2310975492,
0.1531070322,
0.2232790291,
-0.1793228984,
-0.2515394688,
-0.3708984554,
0.1215014681,
-0.0856520385,
0.2131277621,
0.0081082908,
-0.0478743054,
-0.0732750595,
0.1985649318,
-0.2597444654,
-0.4831646383,
-0.4174054265,
0.4844005406,
0.3321518898,
-0.2023144066,
-0.0913062319,
0.1243591756,
-0.0094576795,
-0.0002587312,
-0.1325838715,
0.1982096732,
0.1807583869,
0.3041763902,
0.0009505561,
-0.0164920762,
-0.1214541122,
-0.0175777432,
-0.0014498794,
0.2395069748,
-0.0714901611,
0.1017907932,
0.108518213,
-0.264721632,
0.4198531508,
0.0897680297,
0.5018247962,
-0.3040901124,
0.2026569247,
-0.2344893366,
-0.2914102077,
-0.4042405486,
-0.2128190547,
-0.1952729076,
0.2986359298,
0.1747802198,
0.4102657437,
0.2424885929,
-0.2421736568,
0.1144187674,
0.0731973127,
0.1749113351,
0.1492746323,
0.0461607762,
-0.089212127,
-0.0291164778,
-0.619448781,
0.1014877707,
0.0836122409,
0.004427487,
-0.0240108985,
-0.0721823126,
-0.5134980679,
-0.1284176111,
0.1638021022,
0.2362136841,
0.0536449924,
0.4173861146,
-0.1873999536,
-0.0113127166,
-0.034863729,
-0.0060418132,
0.2730379999,
-0.1915697306,
-0.0835700557,
-0.2481809855,
0.1709206551,
-0.1321861446,
0.0614207201,
-0.1478129625,
0.2032232285,
0.46397385,
-0.0967973471,
0.3414818048,
0.0473216064,
0.2152622044,
-0.1106107309,
-0.0845467895,
-0.3863245547,
0.081785351,
-0.1389549375,
0.4932189584,
0.1644177586,
-0.138568148,
-0.2046209276,
0.1893077642,
0.0041490276,
-0.1906443089,
-0.2836732566,
0.3273252547,
-0.0022105309,
0.1383224577,
0.0360357612,
0.0244637467,
0.2087706327,
0.2192704827,
-0.2801862955,
-0.5413849354,
0.3990265131,
-0.2757386267,
-0.0321881175,
-0.2142983079,
0.3366417587,
0.3930226266,
-0.2063712031,
-0.6328856945,
-0.0117068104,
0.3883118927,
-0.1035703719,
-0.4395235777,
0.0690475032,
0.2488583028,
0.2722867727,
-0.0126971416,
-0.1619986445,
0.2330735028,
-0.0640470982,
0.4385648966,
-0.2267674655
]
|
https://github.com/huggingface/datasets/issues/1959 | Bug in skip_rows argument of load_dataset function ? | Hi,
try `skiprows` instead. This part is not properly documented in the docs it seems.
@lhoestq I'll fix this as part of a bigger PR that fixes typos in the docs. | Hello everyone,
I'm quite new to Git so sorry in advance if I'm breaking some ground rules of issues posting... :/
I tried to use the load_dataset function, from Huggingface datasets library, on a csv file using the skip_rows argument described on Huggingface page to skip the first row containing column names
`test_dataset = load_dataset('csv', data_files=['test_wLabel.tsv'], delimiter='\t', column_names=["id", "sentence", "label"], skip_rows=1)`
But I got the following error message
`__init__() got an unexpected keyword argument 'skip_rows'`
Have I used the wrong argument ? Am I missing something or is this a bug ?
Thank you very much for your time,
Best regards,
Arthur | 31 | Bug in skip_rows argument of load_dataset function ?
Hello everyone,
I'm quite new to Git so sorry in advance if I'm breaking some ground rules of issues posting... :/
I tried to use the load_dataset function, from Huggingface datasets library, on a csv file using the skip_rows argument described on Huggingface page to skip the first row containing column names
`test_dataset = load_dataset('csv', data_files=['test_wLabel.tsv'], delimiter='\t', column_names=["id", "sentence", "label"], skip_rows=1)`
But I got the following error message
`__init__() got an unexpected keyword argument 'skip_rows'`
Have I used the wrong argument ? Am I missing something or is this a bug ?
Thank you very much for your time,
Best regards,
Arthur
Hi,
try `skiprows` instead. This part is not properly documented in the docs it seems.
@lhoestq I'll fix this as part of a bigger PR that fixes typos in the docs. | [
0.1184588745,
-0.5157741308,
-0.0002607238,
0.1341338456,
0.2405335307,
0.3162592947,
0.3996221721,
0.1163903475,
0.1897605658,
0.2767885327,
0.3576671183,
0.3101137877,
0.0523398146,
0.1391008049,
0.212282747,
0.0097794868,
0.3222462535,
-0.0971089825,
-0.2278703153,
-0.1425262243,
-0.4665181935,
0.2059865147,
-0.4954795241,
-0.0062193135,
0.0046913568,
0.2700181305,
-0.1468365192,
0.325058043,
0.1460926384,
-0.0820216313,
0.4361986518,
0.0927887782,
0.0622526556,
0.4919846058,
-0.0001279238,
-0.0485202074,
0.0909041539,
-0.1174369156,
-0.2508835793,
-0.2423613816,
-0.0103976149,
0.1511083245,
-0.1586594731,
-0.3461464643,
-0.0797088444,
0.2208738923,
-0.193183139,
-0.3198308349,
0.017209556,
0.2279164791,
0.1132520586,
0.0995624438,
-0.2322338074,
0.013391153,
0.367978096,
0.1688148975,
-0.202868104,
0.5136333108,
0.0749794319,
-0.1312933564,
0.2170033753,
0.3002198637,
-0.2162895799,
0.1819369197,
0.6969506741,
0.2346369326,
-0.0080308374,
0.0670858994,
0.1547788382,
0.1427002102,
0.3508744836,
-0.2583890557,
-0.1454953253,
-0.5243523121,
0.1148114949,
-0.4422196448,
0.3829133213,
-0.075752832,
-0.1903962046,
0.1892318577,
-0.1961866766,
-0.3662990928,
-0.2399331033,
0.1452040225,
0.2731880844,
-0.3786737919,
-0.1079848185,
0.2083942741,
0.6590318084,
0.0167085156,
-0.0740461424,
-0.145029977,
0.1362961531,
0.4119841754,
-0.5192499161,
0.0457277931,
0.2632993162,
0.5026040673,
0.1499713957,
0.1526492238,
-0.0932039395,
-0.4410413802,
0.4102219343,
0.1674042642,
0.1500697732,
-0.0041120071,
0.165100947,
0.2374444157,
0.1703534871,
-0.0576957539,
0.1190754026,
-0.0006028942,
-0.0619616397,
-0.2427826673,
-0.0386943705,
-0.245796904,
0.3429154754,
-0.2678745985,
-0.2375256121,
0.1017671451,
-0.1411717683,
-0.1343107224,
-0.2298792452,
0.361294657,
0.3145489395,
-0.1731254756,
0.0057553253,
0.2405337542,
-0.1080142856,
-0.139480412,
-0.1983850002,
0.1062843129,
-0.0351237431,
0.252830267,
0.0619633012,
-0.4568333924,
0.0769030079,
0.0545501038,
0.3502004445,
-0.0496465489,
-0.0791884735,
-0.0380573459,
-0.019057937,
0.2549312711,
-0.1363021582,
-0.1448652595,
0.2976911664,
0.1185014769,
-0.0435421951,
0.5042289495,
-0.2794046402,
-0.2222586125,
0.0607966594,
-0.0945082381,
-0.1695892215,
0.0945609286,
-0.6603600383,
0.2222949266,
0.1386057287,
-0.2015701085,
0.0344226994,
-0.0063753342,
-0.3262868524,
-0.2263972014,
0.2093481272,
0.5372992158,
-0.5187487602,
-0.3551664054,
0.1034002379,
0.0400550663,
0.0190910213,
-0.0114845522,
-0.0296286289,
0.10797894,
-0.3077187538,
0.2494835854,
-0.0521683246,
-0.2833321989,
-0.1313029677,
0.0714629516,
-0.0621326007,
0.218078196,
0.0261956565,
-0.2533242404,
-0.0204318464,
-0.1669732332,
0.253334105,
0.1141673401,
-0.2502170205,
0.0942133367,
-0.1150973886,
-0.1965748817,
0.3166653812,
0.0250527281,
-0.2964206636,
0.1144843772,
-0.2064063251,
-0.5897090435,
0.3778428733,
-0.0728814155,
-0.1749577522,
0.1832053512,
0.043815162,
0.1798682511,
0.2096751481,
0.0379981957,
-0.6307151914,
0.1086894274,
0.49497208,
-0.0462614074,
-0.0923270434,
-0.2249066234,
-0.2521226406,
-0.0548983216,
0.1884769499,
0.2854730785,
-0.132773608,
0.0781119466,
-0.1239917874,
-0.0686810389,
-0.1948326975,
0.1549470723,
-0.3675635755,
0.1537381113,
-0.4071644247,
0.2684521973,
0.2598709464,
-0.0959237963,
0.0065900888,
-0.0984928012,
0.2952402234,
-0.3557017446,
-0.1320198178,
0.4505610466,
0.2342848182,
-0.0091306614,
0.086864993,
0.0611074828,
-0.1808294058,
0.0192998108,
-0.1605572701,
-0.160067305,
0.1238658354,
-0.0958675817,
-0.1965261251,
0.3076041043,
-0.3604244292,
0.325520128,
-0.2628006637,
-0.0352187492,
-0.0754590333,
-0.0341865271,
-0.0842182413,
-0.1979155988,
0.5240817666,
-0.0061132093,
0.1118526757,
0.0035343766,
-0.5212615132,
-0.2066823393,
0.383084923,
-0.0621212684,
-0.2070293874,
0.3191438615,
0.1557872146,
-0.0262888018,
0.3411842287,
-0.1251761466,
0.5859798193,
0.044935599,
-0.3043899238,
-0.0666453987,
0.1478589177,
-0.0423215255,
0.2223356515,
0.038546294,
0.1551994085,
-0.2557177544,
-0.1896428168,
0.0230877493,
-0.0583358444,
0.1618839055,
0.0453285277,
0.1793261468,
-0.6254631281,
0.2487065792,
-0.0588403568,
-0.1682414562,
0.1682818085,
0.0879589021,
-0.306044966,
-0.2525306642,
-0.0842329338,
0.064974457,
-0.1292806268,
0.2338946462,
-0.2523551285,
0.6201028824,
-0.1122033522,
-0.2239249945,
-0.3348612487,
-0.0328214541,
-0.0588840507,
-0.0667504147,
0.0557748452,
0.1014678627,
0.3207905293,
-0.0589228123,
-0.1396522969,
-0.0037374513,
-0.0733500198,
0.1602300256,
-0.3428146839,
0.3066677153,
0.2471901923,
0.3997297287,
-0.1542537808,
0.1513815075,
0.0548964664,
-0.0476552062,
0.1007011607,
0.0335225463,
0.2533079982,
0.0797875598,
-0.2920958102,
0.0357825831,
-0.1299384832,
-0.0000386549,
0.221391961,
0.019871654,
-0.0376497321,
-0.0669298843,
0.1179809496,
0.0733959824,
-0.0719336867,
-0.0484873503,
-0.3079354763,
-0.6267727613,
0.2409046739,
-0.0264316089,
-0.1951415688,
-0.02369304,
0.3533590138,
-0.031195756,
-0.1339343339,
-0.4656544924,
-0.2469317913,
0.130547002,
0.1517630517,
-0.196988374,
0.0388501361,
0.0503361262,
-0.2450458705,
0.1536442637,
-0.4438822865,
-0.4812512696,
0.0825283974,
0.1209164709,
0.1065654457,
0.4717402458,
0.2879652977,
-0.2733361125,
0.3163414896,
0.146478802,
0.3638209403,
0.3352499008,
-0.167528674,
0.2650375068,
0.0734613836,
-0.3172513843,
0.1638062596,
0.0464832112,
-0.2111840397,
0.1785172671,
0.0041289362,
0.1407556385,
-0.2208396941,
0.085605666,
-0.0104811387,
-0.389654547,
0.0177369192,
-0.2655597925,
-0.0000427024,
0.066714853,
0.2684611082,
-0.4285847247,
-0.0578813963,
-0.0875501931,
0.1557600051,
0.6310107112,
0.0573401079,
-0.07303036,
-0.0804757103,
-0.371091783,
0.3203975856,
0.0968666747,
-0.0332953222,
0.0873860195,
-0.0594172776,
0.1238117069,
0.192456618,
0.970267415,
0.1808312684,
0.1366528571,
0.1105831861,
0.2175791115,
-0.3055568337,
-0.0134118656,
0.0733851716,
0.267216146,
0.3817238212,
0.2656696141,
-0.4033342898,
-0.2226455063,
0.2769916654,
0.2830925882,
-0.0478490181,
-0.3223295808,
-0.3425842226,
-0.1045978665,
-0.3616630733,
0.1127390638,
0.2983871102,
0.0953748152,
-0.377410084,
0.3649783731,
0.2887406647,
-0.1708687395,
0.0014057874,
0.2205379307,
0.2984919548,
-0.3236278296,
-0.0663356632,
0.426528424,
-0.2523585856,
0.4026009738,
0.6713850498,
-0.3897364438,
-0.4563651085,
-0.0650464594,
-0.500756979,
0.5561573505,
0.2915152013,
-0.3470170796,
0.249436751,
-0.0746394023,
0.0408955999,
-0.1202687174,
-0.3268793225,
0.4433288276,
0.0008115765,
-0.1251006424,
-0.357588172,
0.2981076539,
-0.0859071538,
-0.1844007969,
0.426938355,
0.7055800557,
-0.2070477456,
0.0742950439,
-0.4285734296,
0.9876382947,
0.4479186833,
0.1335489601,
0.1885948628,
0.2559271157,
0.544903636,
0.4156983495,
-0.0394426808,
-0.1166795641,
-0.3400417566,
0.0358400904,
-0.043622572,
0.0801739693,
0.1187168509,
-0.1670794934,
0.2674461603,
-0.0833325014,
0.0977664888,
-0.0778847337,
-0.3814983964,
0.3286144137,
-0.0183286108,
-0.21223782,
-0.021396488,
0.1279188842,
0.0291785169,
0.0027432779,
0.076529488,
0.022550147,
-0.4613629878,
-0.3341669738,
-0.0798490271,
-0.1575936377,
-0.3594911993,
0.29293558,
-0.2710044086,
0.258475244,
0.1882326454,
0.079106316,
-0.1033836976,
-0.2842765152,
-0.083842665,
0.0818975791,
0.1955943555,
-0.3937598765,
0.0037562638,
0.1803210229,
0.0484673381,
-0.2307668477,
0.092599608,
-0.1555216014,
-0.4176957607,
0.0138237542,
0.0713842586,
-0.3471811712,
-0.3193705082,
-0.4029916525,
-0.0582876094,
0.3188601732,
-0.2722458243,
-0.0319175087,
0.1824083626,
0.0771121308,
0.0252368934,
-0.010483711,
-0.3415661752,
-0.0579077974,
0.2334400117,
-0.189017117,
-0.1578359306,
0.2290959209,
0.3940142691,
-0.1259289086,
0.0110727381,
-0.0159356799,
0.1251061112,
-0.4891855121,
0.5048873425,
0.4031617641,
0.4566209316,
0.1132586971,
0.1569551528,
0.0025209009,
-0.1316837519,
0.0398233905,
-0.1272896379,
0.02066838,
0.1495548636,
0.2238907665,
0.1502293199,
0.1870163083,
0.0887276158,
0.0358872823,
-0.0848181769,
-0.1349640489,
0.0610039048,
-0.1800461411,
-0.144287318,
0.7343521118,
0.4233569503,
0.2425293624,
-0.1966067255,
-0.0783286467,
0.0659480095,
0.0952813998,
-0.0415144563,
-0.3675374091,
0.2147178352,
-0.0072646909,
-0.0344188362,
0.0490241498,
-0.2043952197,
-0.1229927465,
-0.2577933073,
0.2782378495,
0.0852607861,
0.0525207929,
0.2377351075,
0.391550988,
0.1032227352,
-0.05925145,
0.1123411283,
0.0507624857,
0.2624183297,
-0.1811741292,
0.108792536,
-0.1437570453,
0.0702599064,
-0.0417991541,
-0.0027773771,
0.0403458439,
0.1710274369,
-0.030533826,
-0.1313513219,
0.169852823,
0.0017847008,
0.0675757676,
0.3770520985,
-0.3807772994,
0.0984963253,
0.0769973472,
-0.010565294,
-0.3598610759,
-0.1977273822,
0.2949619293,
0.1285372972,
-0.0150990952,
0.2488166988,
-0.2123207599,
0.0362754017,
-0.1478247941,
-0.1595069766,
0.5118708014,
0.0680444315,
0.0086554475,
0.5517327189,
-0.1172772199,
0.2473680228,
0.0751567259,
0.3716815114,
0.2079108208,
0.3752682805,
-0.0928772911,
-0.152513355,
0.0226767361,
0.0332649425,
-0.3393265903,
-0.6127125025,
0.1138677001,
0.4662078321,
-0.4114248157,
0.0233357809,
0.0123156421,
0.3759473264,
0.0782099068,
-0.1980503649,
-0.1014165506,
0.0741261765,
0.0208275039,
-0.1253558993,
-0.2443072796,
0.055477526,
-0.0471788459,
-0.0114516122,
-0.2020470202,
-0.0929238722,
0.6958199739,
-0.1093801782,
0.2166818678,
-0.2644773126,
-0.0623313971,
-0.2002691627,
0.1111039743,
0.0200600233,
-0.0145324366,
-0.0366859771,
0.0475840792,
0.3377987146,
-0.0703437403,
0.3302782476,
-0.0045206305,
0.3747518659,
-0.3745858669,
-0.2005813867,
0.0350317247,
0.0564239994,
0.2205990404,
-0.0823413283,
0.2905305028,
0.1375499666,
0.0074117212,
-0.0456092358,
0.4056412876,
0.259539634,
0.1267184168,
-0.5624562502,
0.2345642,
-0.4512850642,
-0.2537254691,
-0.4652949572,
0.1221999899,
0.0452436656,
0.13856408,
0.1585768014,
0.125573054,
-0.0869321749,
0.0617625155,
0.0072452808,
0.104896456,
0.428170234,
0.2795758247,
0.2275762409,
-0.4540311992,
-0.1966574192,
-0.4089969099,
0.1094146147,
-0.0585407093,
0.1503297985,
0.4332963824,
-0.1026709825,
0.2155165523,
0.0754044279,
0.2592977285,
0.0064721252,
-0.0435532294,
0.1727091372,
-0.1886401474,
-0.5312672257,
-0.2145917565,
0.0049197427,
0.1421574652,
-0.1503655463,
0.1773813665,
-0.0768594444,
-0.1850156784,
-0.2282263488,
0.1627290249,
0.1208618507,
0.1198448092,
0.2785857916,
-0.1667267233,
0.2345070839,
-0.0160356313,
-0.0630967394,
-0.0092436289,
-0.0470899493,
-0.3542244434,
-0.0529598929,
-0.2087930441,
-0.0009170063,
-0.3818290234,
-0.3481459618,
0.0208479576,
0.0150349112,
0.130340457,
0.2017198503,
-0.4272089303,
0.2923901677,
-0.0747932047,
0.0261830445,
-0.1406652927,
-0.0978338718,
-0.1842042506,
0.3183942437,
-0.0623556487,
0.0342242382,
0.8977257013,
-0.2795380354,
-0.1409466416,
-0.3316091001,
0.1682134569,
0.132226795,
-0.5405363441,
-0.6190083623,
-0.0539387874,
0.2388798296,
0.3874326348,
-0.2610634863,
0.5420951247,
-0.3744546473,
0.1921133399,
0.0887777433,
0.4205943346,
0.1564858109,
-0.0732057467,
0.2644813955,
-0.2102509439
]
|
https://github.com/huggingface/datasets/issues/1957 | [request] make load_metric api intutive | I agree with this proposal. IMO, `num_process` can also be misleading without reading the docs because this option may seem to leverage `multiprocessing` to compute the final result, which is not the case.
@lhoestq @albertvillanova Are you OK with breaking the API for v2.0 and renaming the params as follows:
* `num_process` -> `world_size`
* `process_id` -> `rank` | ```
metric = load_metric('glue', 'mrpc', num_process=num_process, process_id=rank)
```
May I suggest that `num_process` is confusing as it's singular yet expects a plural value and either
* be deprecated in favor of `num_processes` which is more intuitive since it's plural as its expected value
* or even better why not mimic the established dist environment convention for that purpose, which uses `world_size`.
Same for `process_id` - why reinvent the naming and needing to explain that this is **NOT** `PID`, when we have `rank` already. That is:
```
metric = load_metric('glue', 'mrpc', world_size=world_size, rank=rank)
```
This then fits like a glove into the pytorch DDP and alike envs. and we just need to call:
* `dist.get_world_size()`
* `dist.get_rank()`
So it'd be as simple as:
```
metric = load_metric('glue', 'mrpc', world_size=dist.get_world_size(), rank=dist.get_rank())
```
From: https://pytorch.org/docs/stable/distributed.html#torch.distributed.init_process_group
* `world_size (int, optional)` – Number of processes participating in the job. Required if store is specified.
* `rank (int, optional)` – Rank of the current process. Required if store is specified.
And may be an example would be useful, so that the user doesn't even need to think about where to get `dist`:
```
import torch.distributed as dist
if dist.is_initialized():
metric = load_metric(metric_name, world_size=dist.get_world_size(), rank=dist.get_rank())
else:
metric = load_metric(metric_name)
```
I'm aware this is pytorch-centric, but it's better than no examples, IMHO.
Thank you. | 58 | [request] make load_metric api intutive
```
metric = load_metric('glue', 'mrpc', num_process=num_process, process_id=rank)
```
May I suggest that `num_process` is confusing as it's singular yet expects a plural value and either
* be deprecated in favor of `num_processes` which is more intuitive since it's plural as its expected value
* or even better why not mimic the established dist environment convention for that purpose, which uses `world_size`.
Same for `process_id` - why reinvent the naming and needing to explain that this is **NOT** `PID`, when we have `rank` already. That is:
```
metric = load_metric('glue', 'mrpc', world_size=world_size, rank=rank)
```
This then fits like a glove into the pytorch DDP and alike envs. and we just need to call:
* `dist.get_world_size()`
* `dist.get_rank()`
So it'd be as simple as:
```
metric = load_metric('glue', 'mrpc', world_size=dist.get_world_size(), rank=dist.get_rank())
```
From: https://pytorch.org/docs/stable/distributed.html#torch.distributed.init_process_group
* `world_size (int, optional)` – Number of processes participating in the job. Required if store is specified.
* `rank (int, optional)` – Rank of the current process. Required if store is specified.
And may be an example would be useful, so that the user doesn't even need to think about where to get `dist`:
```
import torch.distributed as dist
if dist.is_initialized():
metric = load_metric(metric_name, world_size=dist.get_world_size(), rank=dist.get_rank())
else:
metric = load_metric(metric_name)
```
I'm aware this is pytorch-centric, but it's better than no examples, IMHO.
Thank you.
I agree with this proposal. IMO, `num_process` can also be misleading without reading the docs because this option may seem to leverage `multiprocessing` to compute the final result, which is not the case.
@lhoestq @albertvillanova Are you OK with breaking the API for v2.0 and renaming the params as follows:
* `num_process` -> `world_size`
* `process_id` -> `rank` | [
-0.2996609211,
-0.6217932105,
-0.0776229724,
0.1083437949,
0.3084196746,
-0.2696101367,
0.2294079065,
-0.1367820948,
0.3890975714,
0.4229172468,
0.0213311817,
0.3503682613,
-0.0348374099,
-0.0302776881,
0.1602787375,
-0.3683152199,
-0.1868816465,
-0.0273016021,
-0.164674297,
0.0291899666,
-0.3645566702,
0.1518687904,
0.1370694935,
-0.0766176209,
0.1887558699,
-0.0083094789,
-0.242960155,
0.2895413935,
-0.1673080921,
-0.3411146104,
0.0402997211,
0.1901098043,
0.2309205085,
0.444758445,
-0.0001109431,
-0.2619940639,
0.3390595615,
-0.0637609437,
-0.1221047342,
-0.1723394096,
0.2721026838,
-0.3656265438,
0.2489384413,
-0.5543490052,
-0.0043050563,
-0.3076120615,
-0.1099739373,
-0.3893929422,
0.2032434195,
0.1900096387,
0.1624382734,
0.3462112844,
-0.202992022,
-0.272525847,
-0.2126147896,
0.0790470317,
0.0878198445,
0.4716375768,
0.3056373894,
-0.0791150033,
-0.279140234,
0.0684460476,
-0.0067308466,
0.3619041741,
0.4685910046,
-0.192548275,
0.2268823385,
-0.1646142155,
-0.0561710037,
0.1804478914,
0.0285027511,
-0.4710617661,
-0.1467192769,
-0.0587241687,
0.0025673658,
-0.4908222854,
-0.2148064673,
0.0482714586,
-0.1023440287,
-0.2451881766,
-0.0722154379,
0.2619205415,
-0.1124696732,
0.0435982943,
-0.1519618928,
0.5194907784,
0.2985062897,
0.1412196457,
0.1124736294,
-0.027847331,
-0.0781326368,
0.3278670609,
0.2503593266,
-0.1523737609,
0.0236578099,
0.0118768383,
0.2891307771,
-0.1492073387,
0.1099776924,
0.0584767982,
-0.060559094,
0.0031943058,
0.4320017099,
0.0954113975,
-0.0799055994,
0.5821461678,
0.415623039,
0.1353164166,
0.0209815204,
-0.0717097521,
0.329030782,
0.0846803859,
-0.1590552926,
-0.1949465871,
0.0709059536,
0.3158110976,
-0.1940741241,
0.25817734,
-0.2966827452,
-0.116027616,
0.2728955746,
-0.0043210299,
0.1303613186,
0.3817545772,
0.0406100415,
0.5169623494,
0.3796899021,
0.066550523,
-0.2117375284,
0.1465497017,
-0.1783590168,
0.0976696387,
-0.2084047794,
0.0694905147,
0.0603969097,
0.0524275452,
0.3074316978,
-0.1228349656,
0.5490765572,
0.1260662526,
0.1288951188,
0.2201017141,
0.1849007905,
0.0774386078,
0.0361356474,
-0.356010586,
0.2225918919,
0.1841636896,
-0.4027043879,
0.1343318671,
-0.2860145569,
-0.3159377873,
-0.3449732959,
0.2277894765,
-0.1226513311,
0.0909439325,
0.0562358759,
0.0770128444,
0.1822000891,
-0.1429638118,
0.281172514,
-0.328222245,
-0.3715538681,
-0.1568496078,
0.1360428184,
0.1827223301,
0.1602302939,
-0.3390844762,
-0.1468831003,
-0.078018032,
0.1663431078,
-0.1616569161,
-0.0394163877,
0.1250497103,
-0.0286615938,
-0.3158186078,
0.5189408064,
-0.6049711704,
-0.1945297718,
0.1235756427,
-0.2863486111,
-0.2874845266,
0.1974787414,
0.0818336532,
0.3087365627,
0.143542707,
0.6447017789,
0.1878867447,
-0.0162559934,
0.1422603726,
-0.1649894267,
-0.0740743056,
0.3768075109,
0.1661827415,
0.3307250738,
-0.0120226555,
0.0744013265,
0.0870290995,
0.1635331959,
0.1010097861,
-0.1515134424,
0.1287084073,
-0.3019216061,
-0.2831751108,
0.307154268,
-0.3291249573,
-0.0142457578,
0.1679549664,
-0.3058499694,
0.1413439661,
0.2329648882,
-0.135472998,
-0.3091506958,
0.2216055095,
0.1539149582,
-0.1371803284,
0.1349493265,
-0.1659152061,
-0.1695539802,
-0.411246568,
-0.062221583,
0.0884631649,
-0.2538388073,
-0.176925689,
-0.1828598082,
-0.2055768818,
-0.0950207114,
-0.0085967164,
-0.0983434245,
0.4127921164,
0.2608588934,
-0.0014516073,
-0.0943724215,
0.3666980863,
0.2728060484,
-0.1715858728,
-0.0458255149,
0.0925555378,
0.2742489874,
0.0060967049,
0.194037661,
0.1591894329,
0.034425918,
-0.0879708976,
-0.1798932105,
0.2957859933,
0.0383681543,
0.2941094041,
-0.118719928,
0.0894320905,
-0.1010236144,
0.0579374768,
-0.259945631,
-0.0762707591,
0.4864525497,
-0.1253510416,
0.2077497691,
-0.0540148467,
0.1427977383,
0.115898639,
0.0505119115,
-0.0751277059,
0.1479464769,
0.130456388,
-0.0152273532,
-0.048173774,
0.2583887279,
-0.2569446564,
0.3617881238,
0.3758071363,
0.115203023,
-0.0461872593,
0.0750552043,
-0.0345661119,
0.0635677055,
0.2346674353,
-0.2544099391,
0.1085218936,
0.3793817163,
0.0928831473,
0.0873730108,
-0.1760877669,
-0.1109324396,
-0.0325039178,
-0.2431411296,
0.1439476162,
0.0005423463,
0.095881775,
0.0288907103,
0.0126970699,
-0.2307150066,
-0.1246589795,
0.1564455628,
-0.1582447141,
-0.1292660981,
0.1921061128,
0.1589944065,
0.0108452244,
-0.0683559999,
-0.4272021353,
0.1235511452,
-0.3828863204,
0.1385475099,
0.0611146167,
-0.1422320753,
0.0810652003,
0.7752315402,
-0.1621275395,
0.0876628309,
0.0355839394,
-0.1837144643,
0.0675486326,
0.0370604135,
-0.0689111277,
0.3541461825,
-0.1138851494,
-0.0847161859,
-0.0211580582,
0.1879863739,
-0.3797694445,
-0.1144756749,
0.0281364825,
-0.1338361949,
0.0184240062,
-0.3269917965,
-0.238373071,
-0.3135788739,
-0.4474152327,
0.0177702978,
0.1455113441,
0.299246788,
-0.2101941556,
-0.0062129591,
0.106353201,
0.7234299779,
0.1843059063,
-0.1845298558,
-0.1367194206,
-0.0137759708,
-0.4514070749,
-0.2675209343,
-0.33559829,
-0.2805554271,
0.1279170513,
0.3151452243,
0.0228600837,
-0.7917456627,
0.1179412231,
0.2651686668,
-0.1913301349,
0.1544703245,
0.6531234384,
0.0797271952,
-0.1141344085,
0.0107116988,
-0.3083710968,
0.1073737442,
0.2348544598,
0.2428355515,
0.0255158916,
0.2635999024,
-0.0852604508,
0.851409018,
-0.2622095346,
-0.2585352659,
0.1516801417,
-0.1849408299,
0.1378163397,
0.0602389872,
-0.2283983082,
0.225262776,
0.0644196123,
0.0267914664,
0.1137737259,
-0.0302172191,
-0.0320853479,
-0.1057560518,
-0.099348858,
-0.0197762046,
0.0377595574,
0.1346047968,
-0.3755747676,
0.1047900245,
-0.2959508598,
0.2480206341,
-0.533149302,
0.0492876843,
0.0688622743,
0.0685811713,
0.0340607092,
0.1040163413,
0.2357998341,
-0.1366520971,
-0.3817615211,
0.4732145965,
0.2063325047,
-0.1499039829,
-0.1694087982,
-0.1048386544,
0.0587812774,
-0.1788701713,
0.1618487388,
0.1963599771,
-0.0200231317,
0.1648662984,
-0.2378822267,
-0.5455292463,
0.1361318529,
0.0015693709,
-0.055490125,
0.1567650884,
0.134293586,
-0.5490243435,
-0.1558075398,
-0.0636698455,
0.1057142615,
-0.2480098456,
-0.3230930567,
-0.4743893743,
-0.319830507,
-0.1856479347,
0.2442758083,
0.300750047,
0.3185735047,
-0.1105680689,
0.0374078155,
-0.0467556939,
0.0422148816,
0.116254665,
-0.0217009615,
0.181103453,
-0.1867247671,
-0.2538162172,
0.1647301912,
0.3908277154,
-0.136651814,
0.2152326256,
-0.4884426892,
-0.0056191152,
0.3011587262,
0.2447038889,
0.3069317043,
0.2156600952,
0.1424331367,
-0.2570473254,
-0.0915795639,
0.2753359079,
-0.3367784917,
0.2994118929,
0.0004984442,
-0.2056971192,
-0.1720266044,
-0.3374549448,
0.4561211169,
0.2391219586,
-0.2081017792,
0.5406825542,
-0.3033943474,
-0.1089696363,
0.1935773939,
0.4626362622,
1.0668292046,
-0.1732781678,
0.0877366513,
0.1900909841,
-0.0009451916,
0.0524452105,
-0.1490857899,
0.1214613691,
-0.39520365,
0.0587350354,
0.0329949446,
-0.0821935013,
-0.1925556958,
-0.136600703,
-0.1739971787,
0.3217383027,
-0.1057430431,
-0.2430510819,
0.0629961044,
0.6426701546,
-0.2510348856,
-0.3621613085,
0.2191555202,
0.1733870953,
-0.2723066807,
0.1179623306,
-0.2286919057,
-0.0781040192,
0.1500458121,
-0.3231050074,
-0.1560789794,
-0.1687168926,
-0.342361629,
0.2198068798,
-0.2971650958,
-0.5436688662,
-0.2499729246,
0.3925357759,
-0.1223874465,
-0.0125578856,
-0.0455987044,
0.0933025703,
-0.0744679645,
0.0765801966,
0.0719693825,
-0.0828469321,
0.1289231628,
-0.1390358657,
-0.1329023242,
-0.2113877833,
0.0500321686,
-0.1736237407,
-0.1773969084,
0.3656278849,
0.5547763705,
0.0204267893,
0.298694253,
-0.1986863464,
0.0256299097,
-0.0804294124,
0.1286483705,
0.0585017055,
-0.0522910245,
-0.0074130413,
-0.1072427854,
-0.1656367481,
0.0596740954,
0.2251493186,
0.0687049031,
-0.1991909444,
-0.1873279661,
-0.0731448531,
-0.164070487,
-0.2191669047,
-0.1520025581,
0.0015614736,
0.1314675063,
0.1242599413,
0.3060702384,
-0.4041810334,
-0.029632723,
-0.0882291198,
0.1158986017,
0.1092449427,
-0.0865999758,
-0.1380135268,
-0.4532729387,
0.0717837736,
-0.2051382363,
0.3441697061,
-0.0068316823,
0.0666153654,
-0.0288151316,
0.1498981416,
-0.3758229613,
-0.2040261328,
0.0282803662,
-0.0538711064,
0.1185101941,
-0.1209418848,
-0.0335951895,
0.1508201659,
0.0283052083,
0.1428044736,
-0.1819509417,
-0.2473118603,
-0.2804589272,
0.1508912444,
-0.19194673,
0.2508400381,
0.060221307,
-0.1349520385,
-0.0999634564,
-0.285710752,
0.1106107607,
0.2983305454,
-0.0689358711,
0.1418277621,
0.3190731108,
0.6217060089,
0.2496399283,
0.2615419924,
0.1990232766,
0.2659105957,
-0.3042254746,
0.1441251189,
0.080554761,
-0.0096601099,
0.087245971,
0.0517220311,
0.5917152166,
-0.0358750299,
-0.028440496,
0.3705540597,
-0.2234514207,
-0.0793190673,
0.3993525505,
0.038398277,
-0.2315226048,
0.1632785052,
-0.0654496923,
0.2516816258,
-0.1839855611,
0.1039664224,
-0.1223364919,
0.1002940312,
0.0918403268,
0.3485176265,
-0.0386040658,
-0.0714227334,
0.0643777549,
-0.0112325121,
0.3561612666,
-0.4494187236,
-0.176895082,
-0.0814246014,
0.0368527696,
-0.038813822,
0.4265612662,
0.0023487743,
0.4430690706,
-0.2420226783,
0.0862927362,
0.6796067357,
0.3807471991,
0.1505141109,
0.3188373148,
0.2243937552,
-0.0248776991,
-0.1503744572,
0.2285554856,
-0.03823613,
-0.0322595723,
0.5460730195,
-0.547688067,
-0.0976585522,
-0.4392390847,
0.1955591291,
-0.0405917689,
0.0905775651,
0.1978973597,
0.0271112695,
-0.0477950312,
-0.3294201791,
0.2422086447,
-0.2759288549,
0.3541638553,
0.047271926,
-0.0184017364,
-0.1603858322,
-0.3523420095,
0.2667719424,
0.1545159966,
-0.2697050869,
-0.2063449919,
0.5141231418,
0.0681544617,
-0.1296975464,
0.2488639951,
0.2875159681,
0.2039901316,
-0.580812037,
-0.1110788658,
0.1639270782,
0.0141657069,
-0.2728540599,
0.0357140452,
-0.0617256686,
-0.0575051792,
0.2190717161,
0.2022242248,
-0.2953879535,
-0.1501013786,
-0.0039080833,
-0.1497273296,
0.1113705263,
0.9793524146,
0.0511064157,
-0.0797745436,
0.0048357127,
-0.0000980906,
-0.5089745522,
-0.0724466294,
0.0100407097,
0.1361043155,
0.0035893917,
-0.2984906733,
0.0965982154,
-0.0525271557,
0.129820317,
-0.0091945408,
0.281912148,
-0.296182543,
0.1966515034,
-0.5823723674,
0.2424448729,
0.1818705946,
-0.2044818997,
-0.0096838437,
-0.1303914338,
-0.0534977987,
0.1278101951,
-0.2682490349,
0.140516907,
0.1863750517,
0.249503091,
-0.3666055501,
0.1445799023,
-0.0976878852,
-0.0282104649,
0.2818748355,
-0.1981207579,
-0.2539371252,
-0.2567666173,
0.0719577596,
-0.2342344522,
-0.1441210657,
0.5169380307,
0.4826299548,
0.3421124816,
0.0291159116,
0.5105262399,
-0.1577291191,
0.1667866856,
0.1634894162,
-0.045521792,
-0.2206109613,
0.0539991073,
-0.0183331408,
-0.1724941581,
-0.0493317768,
-0.6163505316,
-0.2653841972,
0.3970377743,
-0.0833110064,
-0.2571676075,
-0.1600155383,
-0.0033509925,
-0.2208798677,
0.0259304792,
-0.2094207555,
0.2214313149,
0.2503815889,
0.0619830228,
0.0116999093,
-0.2442816049,
0.5075546503,
-0.2946257591,
-0.2220192701,
-0.1563033015,
0.409876734,
0.1143224314,
0.2257052213,
-0.2145216763,
0.0355134569,
0.1573476493,
0.1313364953,
-0.0149186235,
0.2028414011,
-0.2440411747,
0.068043083,
-0.2911981046,
0.0060816132,
-0.1216797382,
-0.1687311232,
-0.0974861681,
-0.2714937627
]
|
https://github.com/huggingface/datasets/issues/1957 | [request] make load_metric api intutive | I don't think that's a good idea for 2.0, we may have a new library for metrics anyway.
Note that we will need an API that also makes sense for TF users | ```
metric = load_metric('glue', 'mrpc', num_process=num_process, process_id=rank)
```
May I suggest that `num_process` is confusing as it's singular yet expects a plural value and either
* be deprecated in favor of `num_processes` which is more intuitive since it's plural as its expected value
* or even better why not mimic the established dist environment convention for that purpose, which uses `world_size`.
Same for `process_id` - why reinvent the naming and needing to explain that this is **NOT** `PID`, when we have `rank` already. That is:
```
metric = load_metric('glue', 'mrpc', world_size=world_size, rank=rank)
```
This then fits like a glove into the pytorch DDP and alike envs. and we just need to call:
* `dist.get_world_size()`
* `dist.get_rank()`
So it'd be as simple as:
```
metric = load_metric('glue', 'mrpc', world_size=dist.get_world_size(), rank=dist.get_rank())
```
From: https://pytorch.org/docs/stable/distributed.html#torch.distributed.init_process_group
* `world_size (int, optional)` – Number of processes participating in the job. Required if store is specified.
* `rank (int, optional)` – Rank of the current process. Required if store is specified.
And may be an example would be useful, so that the user doesn't even need to think about where to get `dist`:
```
import torch.distributed as dist
if dist.is_initialized():
metric = load_metric(metric_name, world_size=dist.get_world_size(), rank=dist.get_rank())
else:
metric = load_metric(metric_name)
```
I'm aware this is pytorch-centric, but it's better than no examples, IMHO.
Thank you. | 32 | [request] make load_metric api intutive
```
metric = load_metric('glue', 'mrpc', num_process=num_process, process_id=rank)
```
May I suggest that `num_process` is confusing as it's singular yet expects a plural value and either
* be deprecated in favor of `num_processes` which is more intuitive since it's plural as its expected value
* or even better why not mimic the established dist environment convention for that purpose, which uses `world_size`.
Same for `process_id` - why reinvent the naming and needing to explain that this is **NOT** `PID`, when we have `rank` already. That is:
```
metric = load_metric('glue', 'mrpc', world_size=world_size, rank=rank)
```
This then fits like a glove into the pytorch DDP and alike envs. and we just need to call:
* `dist.get_world_size()`
* `dist.get_rank()`
So it'd be as simple as:
```
metric = load_metric('glue', 'mrpc', world_size=dist.get_world_size(), rank=dist.get_rank())
```
From: https://pytorch.org/docs/stable/distributed.html#torch.distributed.init_process_group
* `world_size (int, optional)` – Number of processes participating in the job. Required if store is specified.
* `rank (int, optional)` – Rank of the current process. Required if store is specified.
And may be an example would be useful, so that the user doesn't even need to think about where to get `dist`:
```
import torch.distributed as dist
if dist.is_initialized():
metric = load_metric(metric_name, world_size=dist.get_world_size(), rank=dist.get_rank())
else:
metric = load_metric(metric_name)
```
I'm aware this is pytorch-centric, but it's better than no examples, IMHO.
Thank you.
I don't think that's a good idea for 2.0, we may have a new library for metrics anyway.
Note that we will need an API that also makes sense for TF users | [
-0.2996609211,
-0.6217932105,
-0.0776229724,
0.1083437949,
0.3084196746,
-0.2696101367,
0.2294079065,
-0.1367820948,
0.3890975714,
0.4229172468,
0.0213311817,
0.3503682613,
-0.0348374099,
-0.0302776881,
0.1602787375,
-0.3683152199,
-0.1868816465,
-0.0273016021,
-0.164674297,
0.0291899666,
-0.3645566702,
0.1518687904,
0.1370694935,
-0.0766176209,
0.1887558699,
-0.0083094789,
-0.242960155,
0.2895413935,
-0.1673080921,
-0.3411146104,
0.0402997211,
0.1901098043,
0.2309205085,
0.444758445,
-0.0001109431,
-0.2619940639,
0.3390595615,
-0.0637609437,
-0.1221047342,
-0.1723394096,
0.2721026838,
-0.3656265438,
0.2489384413,
-0.5543490052,
-0.0043050563,
-0.3076120615,
-0.1099739373,
-0.3893929422,
0.2032434195,
0.1900096387,
0.1624382734,
0.3462112844,
-0.202992022,
-0.272525847,
-0.2126147896,
0.0790470317,
0.0878198445,
0.4716375768,
0.3056373894,
-0.0791150033,
-0.279140234,
0.0684460476,
-0.0067308466,
0.3619041741,
0.4685910046,
-0.192548275,
0.2268823385,
-0.1646142155,
-0.0561710037,
0.1804478914,
0.0285027511,
-0.4710617661,
-0.1467192769,
-0.0587241687,
0.0025673658,
-0.4908222854,
-0.2148064673,
0.0482714586,
-0.1023440287,
-0.2451881766,
-0.0722154379,
0.2619205415,
-0.1124696732,
0.0435982943,
-0.1519618928,
0.5194907784,
0.2985062897,
0.1412196457,
0.1124736294,
-0.027847331,
-0.0781326368,
0.3278670609,
0.2503593266,
-0.1523737609,
0.0236578099,
0.0118768383,
0.2891307771,
-0.1492073387,
0.1099776924,
0.0584767982,
-0.060559094,
0.0031943058,
0.4320017099,
0.0954113975,
-0.0799055994,
0.5821461678,
0.415623039,
0.1353164166,
0.0209815204,
-0.0717097521,
0.329030782,
0.0846803859,
-0.1590552926,
-0.1949465871,
0.0709059536,
0.3158110976,
-0.1940741241,
0.25817734,
-0.2966827452,
-0.116027616,
0.2728955746,
-0.0043210299,
0.1303613186,
0.3817545772,
0.0406100415,
0.5169623494,
0.3796899021,
0.066550523,
-0.2117375284,
0.1465497017,
-0.1783590168,
0.0976696387,
-0.2084047794,
0.0694905147,
0.0603969097,
0.0524275452,
0.3074316978,
-0.1228349656,
0.5490765572,
0.1260662526,
0.1288951188,
0.2201017141,
0.1849007905,
0.0774386078,
0.0361356474,
-0.356010586,
0.2225918919,
0.1841636896,
-0.4027043879,
0.1343318671,
-0.2860145569,
-0.3159377873,
-0.3449732959,
0.2277894765,
-0.1226513311,
0.0909439325,
0.0562358759,
0.0770128444,
0.1822000891,
-0.1429638118,
0.281172514,
-0.328222245,
-0.3715538681,
-0.1568496078,
0.1360428184,
0.1827223301,
0.1602302939,
-0.3390844762,
-0.1468831003,
-0.078018032,
0.1663431078,
-0.1616569161,
-0.0394163877,
0.1250497103,
-0.0286615938,
-0.3158186078,
0.5189408064,
-0.6049711704,
-0.1945297718,
0.1235756427,
-0.2863486111,
-0.2874845266,
0.1974787414,
0.0818336532,
0.3087365627,
0.143542707,
0.6447017789,
0.1878867447,
-0.0162559934,
0.1422603726,
-0.1649894267,
-0.0740743056,
0.3768075109,
0.1661827415,
0.3307250738,
-0.0120226555,
0.0744013265,
0.0870290995,
0.1635331959,
0.1010097861,
-0.1515134424,
0.1287084073,
-0.3019216061,
-0.2831751108,
0.307154268,
-0.3291249573,
-0.0142457578,
0.1679549664,
-0.3058499694,
0.1413439661,
0.2329648882,
-0.135472998,
-0.3091506958,
0.2216055095,
0.1539149582,
-0.1371803284,
0.1349493265,
-0.1659152061,
-0.1695539802,
-0.411246568,
-0.062221583,
0.0884631649,
-0.2538388073,
-0.176925689,
-0.1828598082,
-0.2055768818,
-0.0950207114,
-0.0085967164,
-0.0983434245,
0.4127921164,
0.2608588934,
-0.0014516073,
-0.0943724215,
0.3666980863,
0.2728060484,
-0.1715858728,
-0.0458255149,
0.0925555378,
0.2742489874,
0.0060967049,
0.194037661,
0.1591894329,
0.034425918,
-0.0879708976,
-0.1798932105,
0.2957859933,
0.0383681543,
0.2941094041,
-0.118719928,
0.0894320905,
-0.1010236144,
0.0579374768,
-0.259945631,
-0.0762707591,
0.4864525497,
-0.1253510416,
0.2077497691,
-0.0540148467,
0.1427977383,
0.115898639,
0.0505119115,
-0.0751277059,
0.1479464769,
0.130456388,
-0.0152273532,
-0.048173774,
0.2583887279,
-0.2569446564,
0.3617881238,
0.3758071363,
0.115203023,
-0.0461872593,
0.0750552043,
-0.0345661119,
0.0635677055,
0.2346674353,
-0.2544099391,
0.1085218936,
0.3793817163,
0.0928831473,
0.0873730108,
-0.1760877669,
-0.1109324396,
-0.0325039178,
-0.2431411296,
0.1439476162,
0.0005423463,
0.095881775,
0.0288907103,
0.0126970699,
-0.2307150066,
-0.1246589795,
0.1564455628,
-0.1582447141,
-0.1292660981,
0.1921061128,
0.1589944065,
0.0108452244,
-0.0683559999,
-0.4272021353,
0.1235511452,
-0.3828863204,
0.1385475099,
0.0611146167,
-0.1422320753,
0.0810652003,
0.7752315402,
-0.1621275395,
0.0876628309,
0.0355839394,
-0.1837144643,
0.0675486326,
0.0370604135,
-0.0689111277,
0.3541461825,
-0.1138851494,
-0.0847161859,
-0.0211580582,
0.1879863739,
-0.3797694445,
-0.1144756749,
0.0281364825,
-0.1338361949,
0.0184240062,
-0.3269917965,
-0.238373071,
-0.3135788739,
-0.4474152327,
0.0177702978,
0.1455113441,
0.299246788,
-0.2101941556,
-0.0062129591,
0.106353201,
0.7234299779,
0.1843059063,
-0.1845298558,
-0.1367194206,
-0.0137759708,
-0.4514070749,
-0.2675209343,
-0.33559829,
-0.2805554271,
0.1279170513,
0.3151452243,
0.0228600837,
-0.7917456627,
0.1179412231,
0.2651686668,
-0.1913301349,
0.1544703245,
0.6531234384,
0.0797271952,
-0.1141344085,
0.0107116988,
-0.3083710968,
0.1073737442,
0.2348544598,
0.2428355515,
0.0255158916,
0.2635999024,
-0.0852604508,
0.851409018,
-0.2622095346,
-0.2585352659,
0.1516801417,
-0.1849408299,
0.1378163397,
0.0602389872,
-0.2283983082,
0.225262776,
0.0644196123,
0.0267914664,
0.1137737259,
-0.0302172191,
-0.0320853479,
-0.1057560518,
-0.099348858,
-0.0197762046,
0.0377595574,
0.1346047968,
-0.3755747676,
0.1047900245,
-0.2959508598,
0.2480206341,
-0.533149302,
0.0492876843,
0.0688622743,
0.0685811713,
0.0340607092,
0.1040163413,
0.2357998341,
-0.1366520971,
-0.3817615211,
0.4732145965,
0.2063325047,
-0.1499039829,
-0.1694087982,
-0.1048386544,
0.0587812774,
-0.1788701713,
0.1618487388,
0.1963599771,
-0.0200231317,
0.1648662984,
-0.2378822267,
-0.5455292463,
0.1361318529,
0.0015693709,
-0.055490125,
0.1567650884,
0.134293586,
-0.5490243435,
-0.1558075398,
-0.0636698455,
0.1057142615,
-0.2480098456,
-0.3230930567,
-0.4743893743,
-0.319830507,
-0.1856479347,
0.2442758083,
0.300750047,
0.3185735047,
-0.1105680689,
0.0374078155,
-0.0467556939,
0.0422148816,
0.116254665,
-0.0217009615,
0.181103453,
-0.1867247671,
-0.2538162172,
0.1647301912,
0.3908277154,
-0.136651814,
0.2152326256,
-0.4884426892,
-0.0056191152,
0.3011587262,
0.2447038889,
0.3069317043,
0.2156600952,
0.1424331367,
-0.2570473254,
-0.0915795639,
0.2753359079,
-0.3367784917,
0.2994118929,
0.0004984442,
-0.2056971192,
-0.1720266044,
-0.3374549448,
0.4561211169,
0.2391219586,
-0.2081017792,
0.5406825542,
-0.3033943474,
-0.1089696363,
0.1935773939,
0.4626362622,
1.0668292046,
-0.1732781678,
0.0877366513,
0.1900909841,
-0.0009451916,
0.0524452105,
-0.1490857899,
0.1214613691,
-0.39520365,
0.0587350354,
0.0329949446,
-0.0821935013,
-0.1925556958,
-0.136600703,
-0.1739971787,
0.3217383027,
-0.1057430431,
-0.2430510819,
0.0629961044,
0.6426701546,
-0.2510348856,
-0.3621613085,
0.2191555202,
0.1733870953,
-0.2723066807,
0.1179623306,
-0.2286919057,
-0.0781040192,
0.1500458121,
-0.3231050074,
-0.1560789794,
-0.1687168926,
-0.342361629,
0.2198068798,
-0.2971650958,
-0.5436688662,
-0.2499729246,
0.3925357759,
-0.1223874465,
-0.0125578856,
-0.0455987044,
0.0933025703,
-0.0744679645,
0.0765801966,
0.0719693825,
-0.0828469321,
0.1289231628,
-0.1390358657,
-0.1329023242,
-0.2113877833,
0.0500321686,
-0.1736237407,
-0.1773969084,
0.3656278849,
0.5547763705,
0.0204267893,
0.298694253,
-0.1986863464,
0.0256299097,
-0.0804294124,
0.1286483705,
0.0585017055,
-0.0522910245,
-0.0074130413,
-0.1072427854,
-0.1656367481,
0.0596740954,
0.2251493186,
0.0687049031,
-0.1991909444,
-0.1873279661,
-0.0731448531,
-0.164070487,
-0.2191669047,
-0.1520025581,
0.0015614736,
0.1314675063,
0.1242599413,
0.3060702384,
-0.4041810334,
-0.029632723,
-0.0882291198,
0.1158986017,
0.1092449427,
-0.0865999758,
-0.1380135268,
-0.4532729387,
0.0717837736,
-0.2051382363,
0.3441697061,
-0.0068316823,
0.0666153654,
-0.0288151316,
0.1498981416,
-0.3758229613,
-0.2040261328,
0.0282803662,
-0.0538711064,
0.1185101941,
-0.1209418848,
-0.0335951895,
0.1508201659,
0.0283052083,
0.1428044736,
-0.1819509417,
-0.2473118603,
-0.2804589272,
0.1508912444,
-0.19194673,
0.2508400381,
0.060221307,
-0.1349520385,
-0.0999634564,
-0.285710752,
0.1106107607,
0.2983305454,
-0.0689358711,
0.1418277621,
0.3190731108,
0.6217060089,
0.2496399283,
0.2615419924,
0.1990232766,
0.2659105957,
-0.3042254746,
0.1441251189,
0.080554761,
-0.0096601099,
0.087245971,
0.0517220311,
0.5917152166,
-0.0358750299,
-0.028440496,
0.3705540597,
-0.2234514207,
-0.0793190673,
0.3993525505,
0.038398277,
-0.2315226048,
0.1632785052,
-0.0654496923,
0.2516816258,
-0.1839855611,
0.1039664224,
-0.1223364919,
0.1002940312,
0.0918403268,
0.3485176265,
-0.0386040658,
-0.0714227334,
0.0643777549,
-0.0112325121,
0.3561612666,
-0.4494187236,
-0.176895082,
-0.0814246014,
0.0368527696,
-0.038813822,
0.4265612662,
0.0023487743,
0.4430690706,
-0.2420226783,
0.0862927362,
0.6796067357,
0.3807471991,
0.1505141109,
0.3188373148,
0.2243937552,
-0.0248776991,
-0.1503744572,
0.2285554856,
-0.03823613,
-0.0322595723,
0.5460730195,
-0.547688067,
-0.0976585522,
-0.4392390847,
0.1955591291,
-0.0405917689,
0.0905775651,
0.1978973597,
0.0271112695,
-0.0477950312,
-0.3294201791,
0.2422086447,
-0.2759288549,
0.3541638553,
0.047271926,
-0.0184017364,
-0.1603858322,
-0.3523420095,
0.2667719424,
0.1545159966,
-0.2697050869,
-0.2063449919,
0.5141231418,
0.0681544617,
-0.1296975464,
0.2488639951,
0.2875159681,
0.2039901316,
-0.580812037,
-0.1110788658,
0.1639270782,
0.0141657069,
-0.2728540599,
0.0357140452,
-0.0617256686,
-0.0575051792,
0.2190717161,
0.2022242248,
-0.2953879535,
-0.1501013786,
-0.0039080833,
-0.1497273296,
0.1113705263,
0.9793524146,
0.0511064157,
-0.0797745436,
0.0048357127,
-0.0000980906,
-0.5089745522,
-0.0724466294,
0.0100407097,
0.1361043155,
0.0035893917,
-0.2984906733,
0.0965982154,
-0.0525271557,
0.129820317,
-0.0091945408,
0.281912148,
-0.296182543,
0.1966515034,
-0.5823723674,
0.2424448729,
0.1818705946,
-0.2044818997,
-0.0096838437,
-0.1303914338,
-0.0534977987,
0.1278101951,
-0.2682490349,
0.140516907,
0.1863750517,
0.249503091,
-0.3666055501,
0.1445799023,
-0.0976878852,
-0.0282104649,
0.2818748355,
-0.1981207579,
-0.2539371252,
-0.2567666173,
0.0719577596,
-0.2342344522,
-0.1441210657,
0.5169380307,
0.4826299548,
0.3421124816,
0.0291159116,
0.5105262399,
-0.1577291191,
0.1667866856,
0.1634894162,
-0.045521792,
-0.2206109613,
0.0539991073,
-0.0183331408,
-0.1724941581,
-0.0493317768,
-0.6163505316,
-0.2653841972,
0.3970377743,
-0.0833110064,
-0.2571676075,
-0.1600155383,
-0.0033509925,
-0.2208798677,
0.0259304792,
-0.2094207555,
0.2214313149,
0.2503815889,
0.0619830228,
0.0116999093,
-0.2442816049,
0.5075546503,
-0.2946257591,
-0.2220192701,
-0.1563033015,
0.409876734,
0.1143224314,
0.2257052213,
-0.2145216763,
0.0355134569,
0.1573476493,
0.1313364953,
-0.0149186235,
0.2028414011,
-0.2440411747,
0.068043083,
-0.2911981046,
0.0060816132,
-0.1216797382,
-0.1687311232,
-0.0974861681,
-0.2714937627
]
|
https://github.com/huggingface/datasets/issues/1956 | [distributed env] potentially unsafe parallel execution | You can pass the same `experiment_id` for all the metrics of the same group, and use another `experiment_id` for the other groups.
Maybe we can add an environment variable that sets the default value for `experiment_id` ? What do you think ? | ```
metric = load_metric('glue', 'mrpc', num_process=num_process, process_id=rank)
```
presumes that there is only one set of parallel processes running - and will intermittently fail if you have multiple sets running as they will surely overwrite each other. Similar to https://github.com/huggingface/datasets/issues/1942 (but for a different reason).
That's why dist environments use some unique to a group identifier so that each group is dealt with separately.
e.g. the env-way of pytorch dist syncing is done with a unique per set `MASTER_ADDRESS+MASTER_PORT`
So ideally this interface should ask for a shared secret to do the right thing.
I'm not reporting an immediate need, but am only flagging that this will hit someone down the road.
This problem can be remedied by adding a new optional `shared_secret` option, which can then be used to differentiate different groups of processes. and this secret should be part of the file lock name and the experiment.
Thank you | 42 | [distributed env] potentially unsafe parallel execution
```
metric = load_metric('glue', 'mrpc', num_process=num_process, process_id=rank)
```
presumes that there is only one set of parallel processes running - and will intermittently fail if you have multiple sets running as they will surely overwrite each other. Similar to https://github.com/huggingface/datasets/issues/1942 (but for a different reason).
That's why dist environments use some unique to a group identifier so that each group is dealt with separately.
e.g. the env-way of pytorch dist syncing is done with a unique per set `MASTER_ADDRESS+MASTER_PORT`
So ideally this interface should ask for a shared secret to do the right thing.
I'm not reporting an immediate need, but am only flagging that this will hit someone down the road.
This problem can be remedied by adding a new optional `shared_secret` option, which can then be used to differentiate different groups of processes. and this secret should be part of the file lock name and the experiment.
Thank you
You can pass the same `experiment_id` for all the metrics of the same group, and use another `experiment_id` for the other groups.
Maybe we can add an environment variable that sets the default value for `experiment_id` ? What do you think ? | [
-0.3388578296,
-0.4435222745,
-0.0121533908,
0.0259140898,
-0.0003200319,
-0.1188518181,
0.4191742837,
-0.0220881831,
0.694543004,
0.334756881,
-0.0704571903,
0.2575862706,
0.020857811,
0.0921891034,
-0.1329858452,
-0.0292747766,
-0.06194143,
-0.1000038311,
-0.1330745667,
-0.1348374039,
-0.3230050504,
0.1404810548,
-0.1086488664,
0.0332357883,
-0.111566335,
-0.0510079749,
-0.2452468127,
0.2668412924,
-0.2192955464,
-0.3962014019,
-0.0470969789,
0.6610615849,
-0.040151,
0.3781708777,
-0.0001052099,
-0.0733859539,
0.140361771,
-0.1786131263,
0.1255242676,
-0.0316812992,
-0.000416295,
-0.2217448205,
0.1787688434,
-0.5844718218,
0.1618597955,
-0.3749355078,
0.1602334082,
-0.6200053692,
0.5229045749,
-0.0920471326,
0.182814464,
0.4089606106,
-0.3570385575,
-0.1778222769,
0.0755403787,
-0.0741302446,
0.0622764565,
0.428599,
0.4382113814,
-0.1648912132,
-0.3774006367,
0.0479231142,
-0.0095324116,
0.4112267494,
0.5238330364,
0.1457097083,
0.1289580166,
-0.2262360752,
-0.3590706885,
0.5437491536,
0.062151555,
-0.0059186243,
-0.2175005823,
-0.0875977501,
-0.0445220396,
-0.2018628865,
0.0316548795,
0.073078163,
-0.2755063772,
0.0701170787,
-0.0908006206,
0.1095004529,
-0.2334238738,
-0.0166138709,
0.1179819852,
0.4391718209,
0.1565181762,
0.3073609173,
0.4072217345,
0.1744004637,
-0.5871927738,
0.322915405,
-0.1573160738,
-0.1374910027,
-0.2163518965,
-0.0715377405,
-0.134501189,
-0.2474489808,
0.2707520425,
0.2180185169,
-0.1847602725,
-0.0347355269,
0.501208365,
0.2264461368,
0.1733482331,
0.222548753,
-0.0145001514,
0.292763412,
0.3371620774,
0.0463935882,
0.0130739342,
0.1034730524,
0.2154527754,
-0.086606279,
0.0407123268,
0.0291972943,
0.0415122993,
-0.0102340616,
-0.4665407538,
0.1799822152,
0.1296784282,
-0.0897997394,
0.1274871528,
0.234956637,
0.2761307061,
-0.1984881908,
0.2240093946,
0.0891114101,
-0.2850963175,
0.2397982925,
-0.1558062732,
-0.0192011278,
-0.3922470212,
0.0479356758,
-0.0023126896,
0.235463053,
0.3477463424,
0.0911863446,
0.3725246191,
0.0711544678,
0.1579838097,
0.1498431116,
0.1748497635,
0.1164056733,
-0.0279611964,
-0.1533219665,
0.1613995433,
-0.0924460515,
-0.1918705255,
-0.2538032532,
-0.2990010679,
-0.1078146771,
-0.0957442597,
0.2104450911,
-0.4288646281,
0.179406777,
0.3127838671,
-0.0441893674,
-0.0737613663,
-0.1354022026,
0.441211313,
-0.139759019,
-0.0608985908,
-0.1054957435,
0.0603922829,
0.5278869271,
0.1167063192,
-0.3138537407,
0.1733953059,
-0.2131506652,
-0.0626811087,
0.2512883544,
-0.0731379241,
-0.1633246243,
-0.1726192087,
0.045132678,
0.1839678138,
-0.5704571605,
-0.4551758468,
0.22675246,
-0.4629923999,
-0.1833775043,
0.5246548653,
0.399215579,
0.491935432,
-0.1816744208,
0.1842800528,
0.1285000294,
-0.0225711595,
-0.0210044198,
-0.2199544162,
-0.2132082433,
0.2055387199,
0.1335553974,
-0.0407904126,
-0.029986931,
0.1842955947,
0.0449085794,
0.3806381226,
0.0599328987,
0.049430605,
0.0249997303,
0.2068836838,
0.0262047928,
0.1731613427,
0.0735590458,
-0.0773375705,
0.1987994611,
-0.255199194,
0.0786853135,
0.1668829173,
-0.2616328299,
0.0597447194,
-0.1807134897,
-0.3017636836,
-0.3694491386,
0.1339280307,
0.1007093191,
-0.4253529608,
-0.2251146138,
-0.1763159931,
0.4126906693,
-0.002603194,
-0.0783251226,
-0.1774894446,
-0.1190527454,
-0.2866605818,
0.0046237819,
-0.0351306945,
0.1059897095,
0.1628839523,
-0.1626183242,
-0.183540538,
0.3546158373,
0.3039298952,
0.109246403,
0.33553195,
0.1854083985,
0.1129210442,
-0.1525602937,
0.1541461796,
0.0319653004,
0.0296031535,
0.0468522422,
-0.1756989211,
0.3792438209,
-0.1660753191,
-0.0425316431,
-0.1739492863,
0.205209896,
-0.0407577269,
-0.1759889275,
-0.3004974425,
-0.1131734252,
0.5896610022,
0.2008985132,
0.1878552586,
0.0096998932,
-0.0725956112,
0.0389046259,
0.0934054926,
0.1792061329,
0.0130478684,
-0.1290570199,
0.2640556395,
0.0338385068,
0.0100355605,
0.3682818711,
0.5299091339,
0.2942914367,
-0.0035119657,
0.2998713851,
-0.0220681131,
-0.2826880813,
-0.0080958428,
0.0303521845,
-0.1510359049,
0.1437178105,
-0.0427938104,
0.0623153001,
-0.1387622505,
-0.2496975958,
0.1067964509,
0.0581835061,
-0.3008230627,
0.2420986742,
-0.092812635,
0.2503353655,
-0.0295379236,
-0.1750535816,
-0.1717951745,
-0.3238008916,
0.2914565802,
0.0818374231,
-0.452172488,
0.1862104833,
0.0592275038,
0.3545638919,
-0.1497489959,
0.078010425,
-0.0674724132,
-0.3504400253,
-0.0287412349,
0.0338267609,
0.0693553239,
0.2826055586,
0.7376533151,
-0.0895641595,
-0.1716848016,
-0.3883164823,
-0.1675386578,
-0.0548318289,
-0.2927384079,
0.5755817294,
0.3770568371,
0.1170967519,
-0.1565518975,
-0.2018890977,
0.1285160929,
-0.4124996364,
-0.154450193,
-0.1241053417,
0.0733179897,
-0.287805438,
-0.3132180572,
-0.4251737595,
-0.3864905834,
-0.3381043375,
0.1782752872,
-0.1811825335,
0.1448947489,
0.0601227246,
-0.3515414596,
-0.0152210351,
0.292881012,
-0.1243957132,
-0.4747363925,
-0.6132942438,
0.1247874424,
-0.3289966583,
-0.2105764896,
-0.0699181408,
0.2135723531,
-0.1581746042,
0.2809074521,
-0.241275534,
-0.7833876014,
0.1669146717,
0.1982200742,
-0.1628028601,
0.0633634999,
0.2734368145,
-0.0901561454,
-0.1465456039,
-0.1769040674,
0.018281877,
0.5482851267,
-0.052514907,
0.1894312054,
-0.1148565039,
0.0894956365,
0.008357482,
0.5683795214,
0.3369276822,
0.012904196,
0.4058043063,
-0.1494740546,
0.4014565647,
0.1018190533,
-0.2884705067,
0.312634021,
0.2290751934,
-0.2266400009,
0.050981652,
-0.2824376523,
0.2149923891,
-0.0292826127,
-0.1109168231,
0.1569551528,
-0.3881768882,
-0.1111628935,
0.0122719668,
0.0890300646,
-0.2054056525,
0.1362083554,
0.0220757741,
-0.0265205279,
0.0318307206,
0.5858515501,
-0.0124930851,
0.1177794561,
-0.0797204897,
-0.1668655425,
-0.3578243256,
0.4702191949,
-0.0713935122,
0.4873968661,
-0.3471036851,
0.0088336794,
0.1524149477,
-0.0133305723,
0.5068030953,
0.0612409674,
0.1467697322,
-0.0510438047,
0.0666102618,
-0.0926594064,
-0.2801855803,
-0.2568097711,
-0.0583580881,
0.2295816988,
0.1282975078,
-0.4007205963,
-0.3233206272,
0.0673334077,
-0.1687121242,
-0.332030952,
-0.4579874575,
-0.4411282837,
-0.285335958,
-0.3242385685,
0.2526416779,
0.0713430196,
-0.1816560179,
-0.021464562,
0.0082047535,
0.1470402777,
0.247108385,
0.0978486538,
0.0833588764,
0.1141773015,
-0.1949962676,
0.1316141039,
0.4105846882,
0.1862627119,
0.0891106278,
0.2787955403,
-0.2423748821,
0.0301725883,
0.1517164409,
-0.1628012508,
0.0851591676,
0.3966119587,
0.0636258721,
-0.0428080447,
0.0545018911,
0.337320447,
-0.3178877532,
0.0252184868,
0.3418804407,
0.0325720757,
-0.1114490554,
-0.0825198367,
0.1174814403,
0.1140536219,
-0.2343800068,
0.3709226549,
0.0198113844,
-0.1443084478,
0.304214865,
0.1867204905,
0.9030950665,
0.0311158504,
0.2280944139,
0.217377305,
0.0732169971,
0.1633864194,
-0.0176223628,
0.0419675298,
-0.3961946964,
-0.3439329863,
-0.1805067807,
-0.1347694099,
0.2987574041,
-0.2991394997,
-0.3444772065,
0.3026094735,
-0.0014976843,
-0.2954223156,
-0.452396512,
0.4312304556,
-0.3331826329,
-0.193841517,
0.2419087291,
0.1094017401,
-0.0754099861,
0.2564890981,
-0.0621124133,
-0.2436825186,
0.0105549283,
-0.3213075399,
-0.3890613019,
-0.2424221337,
0.0219554827,
0.2903731465,
-0.0039324532,
-0.1580264866,
-0.1069825143,
-0.0413959622,
0.0664161518,
0.2461609244,
-0.1328766346,
0.2183260471,
-0.2534843087,
0.0385651104,
0.0761464611,
-0.3603295684,
0.3276646137,
-0.1171878725,
-0.5082362294,
-0.1755797118,
0.1454009861,
-0.2381668538,
0.0332748629,
0.1399683356,
0.355723232,
-0.0296966229,
0.1975515336,
-0.1510793567,
-0.2765432894,
-0.1418731064,
0.1220528334,
0.2428268641,
-0.279132545,
0.3559332788,
-0.1460067928,
-0.3269095719,
0.1301740855,
0.1035872176,
-0.048268836,
-0.057015989,
0.2107542753,
-0.2135889381,
-0.0768896267,
-0.2594356835,
0.0709313825,
-0.0081097065,
0.0050345431,
0.0122505734,
0.1308853328,
-0.2050808668,
0.0797920004,
0.1393647939,
0.330121994,
0.1170084327,
0.060525652,
-0.4716095328,
-0.2771270573,
0.0881799012,
-0.4826112986,
0.4530037642,
0.0873673633,
0.0094036087,
0.0200226381,
0.2794269025,
-0.3884775043,
0.0165667236,
-0.3601017296,
-0.148297146,
0.3039813936,
-0.0586703978,
0.0161727704,
-0.0914912,
0.0403941236,
0.1112423465,
-0.5826277137,
-0.1632359624,
-0.3143567741,
0.1723042876,
-0.1240757331,
-0.035365887,
0.0486165546,
-0.498581171,
-0.0422140732,
-0.0605110973,
0.1797477603,
0.4235540628,
-0.1383517534,
0.0699022338,
0.0984757394,
0.2840333283,
0.1419791579,
0.2620128095,
0.1505046487,
-0.1604099572,
-0.2000435144,
0.1210673973,
-0.2418048382,
-0.0977049619,
-0.1702807546,
0.1415650994,
0.4924522638,
0.2272936404,
-0.1555063725,
0.2823542356,
-0.1449466944,
-0.0772125199,
0.4140521586,
0.3748759329,
-0.2612326443,
-0.1307840943,
0.0538162142,
0.2483969778,
-0.2528646588,
-0.0649076328,
0.1949885637,
0.3159151375,
0.138404265,
0.3598710299,
0.3957144022,
-0.0030838929,
-0.1721450835,
0.0037231457,
0.3735215664,
-0.1775871366,
0.2339083552,
-0.244397074,
-0.1510742605,
0.160831064,
0.3821862042,
0.0139731923,
0.3264165521,
0.0110325813,
-0.2467037886,
0.3727158308,
0.360727042,
0.0910367891,
0.0966885015,
-0.0279843248,
0.0726909563,
-0.1473213881,
-0.0689116791,
0.084878765,
0.238933444,
0.6212900877,
-0.5725151896,
-0.1553122997,
-0.2476171404,
0.3540138006,
-0.1840918511,
-0.0106132179,
0.1967909932,
-0.1030417681,
-0.1526863426,
-0.1799847931,
0.2436831892,
-0.035268113,
0.2975536287,
0.2469143122,
0.1061951667,
-0.2136849165,
-0.1666725874,
0.1843447685,
0.1577889323,
-0.4586445391,
-0.1551934034,
0.3142163157,
-0.1442613155,
0.4445006847,
0.3467244208,
0.2973740399,
0.1290788949,
-0.2095438838,
-0.0285072904,
0.0848276243,
0.1700478047,
-0.1163834035,
0.1652919948,
-0.0131251542,
-0.301889509,
0.2105091363,
0.1445438564,
-0.1336322874,
0.0790036395,
0.1094840094,
-0.0485222265,
-0.0589638948,
0.5235518217,
0.0981116965,
0.0814317018,
0.0804446414,
0.1009467989,
-0.6264944673,
0.1366880834,
0.0718841553,
0.0941771045,
0.2018314451,
-0.3069218099,
0.1171846911,
-0.0118222591,
0.376724571,
0.1390981376,
0.086815685,
-0.4619700611,
0.1721453071,
-0.7986825705,
0.5472495556,
0.0534064323,
-0.4315547049,
-0.016055584,
0.044231277,
0.0583801344,
0.1264326572,
0.3685827553,
0.3651023507,
-0.0372742526,
0.3127582371,
-0.5110433698,
0.2197712511,
0.1067206636,
-0.1656026095,
0.2129304558,
-0.2172720432,
0.1808340102,
-0.0124269649,
0.0676131621,
0.070223175,
0.0709809661,
0.2476882935,
0.049905356,
0.2937146127,
0.0575812273,
0.5136566758,
-0.0202784389,
-0.0191465784,
-0.1074811369,
-0.0272797272,
-0.2270099074,
-0.3902617693,
0.1994393319,
0.0170683507,
-0.2086762041,
-0.1834442914,
-0.4254196286,
0.1746607125,
-0.2887330651,
-0.0300680101,
-0.3006604314,
0.2215656936,
0.0388801396,
0.130300656,
0.0145913949,
0.3273492157,
0.2044259608,
0.1432472765,
-0.1279268563,
-0.0846335888,
0.5487346649,
-0.2959802151,
-0.1867411584,
-0.2512256205,
0.4572097659,
0.1084876433,
0.1357614696,
-0.456859678,
0.0080924444,
0.0233042426,
-0.0894660503,
-0.3460147977,
0.1537270546,
-0.2121053487,
-0.1914071888,
-0.0443380959,
0.0226716716,
0.0970134512,
-0.4199604094,
0.2008334696,
-0.1824048907
]
|
https://github.com/huggingface/datasets/issues/1956 | [distributed env] potentially unsafe parallel execution | Ah, you're absolutely correct, @lhoestq - it's exactly the equivalent of the shared secret. Thank you! | ```
metric = load_metric('glue', 'mrpc', num_process=num_process, process_id=rank)
```
presumes that there is only one set of parallel processes running - and will intermittently fail if you have multiple sets running as they will surely overwrite each other. Similar to https://github.com/huggingface/datasets/issues/1942 (but for a different reason).
That's why dist environments use some unique to a group identifier so that each group is dealt with separately.
e.g. the env-way of pytorch dist syncing is done with a unique per set `MASTER_ADDRESS+MASTER_PORT`
So ideally this interface should ask for a shared secret to do the right thing.
I'm not reporting an immediate need, but am only flagging that this will hit someone down the road.
This problem can be remedied by adding a new optional `shared_secret` option, which can then be used to differentiate different groups of processes. and this secret should be part of the file lock name and the experiment.
Thank you | 16 | [distributed env] potentially unsafe parallel execution
```
metric = load_metric('glue', 'mrpc', num_process=num_process, process_id=rank)
```
presumes that there is only one set of parallel processes running - and will intermittently fail if you have multiple sets running as they will surely overwrite each other. Similar to https://github.com/huggingface/datasets/issues/1942 (but for a different reason).
That's why dist environments use some unique to a group identifier so that each group is dealt with separately.
e.g. the env-way of pytorch dist syncing is done with a unique per set `MASTER_ADDRESS+MASTER_PORT`
So ideally this interface should ask for a shared secret to do the right thing.
I'm not reporting an immediate need, but am only flagging that this will hit someone down the road.
This problem can be remedied by adding a new optional `shared_secret` option, which can then be used to differentiate different groups of processes. and this secret should be part of the file lock name and the experiment.
Thank you
Ah, you're absolutely correct, @lhoestq - it's exactly the equivalent of the shared secret. Thank you! | [
-0.2438626289,
-0.5634173751,
-0.0252404492,
-0.0789235681,
-0.088331379,
-0.0465873741,
0.4310411215,
-0.0947356373,
0.6884128451,
0.3382454216,
-0.0324465074,
0.2416060269,
0.0294991489,
0.0651076138,
-0.0662355497,
0.0515501313,
-0.0137191424,
-0.0775891468,
-0.2323585749,
-0.1388346851,
-0.2581668794,
0.1642915756,
-0.1347394884,
0.1182619855,
-0.1310549527,
-0.0675273091,
-0.293854177,
0.3518730104,
-0.2069531679,
-0.3734538257,
-0.0511101335,
0.5885391235,
-0.1115159169,
0.3470312655,
-0.0001025435,
-0.029089652,
0.2390751243,
-0.184021309,
0.0937752947,
-0.0225163326,
-0.0063963458,
-0.2147798538,
0.2390900701,
-0.6090912819,
0.2548006773,
-0.31782341,
0.3465066254,
-0.5903223753,
0.4716383219,
-0.1917704344,
0.2114201486,
0.4353038669,
-0.238369897,
-0.21038872,
0.0767692849,
-0.1481728852,
0.0649377182,
0.3659193814,
0.4074767828,
-0.1650008708,
-0.3139854372,
-0.0395434871,
0.0435986035,
0.3771085739,
0.5423665643,
0.2160795927,
-0.0078242067,
-0.3159534037,
-0.3685429692,
0.544993639,
0.0689925775,
-0.0175679978,
-0.1552129984,
-0.0432233997,
-0.1245839894,
-0.0624337085,
0.0405216441,
0.0801770687,
-0.2795662582,
0.0957412645,
-0.0633942112,
0.2075609565,
-0.1429087073,
-0.0452655517,
0.0962149799,
0.4674389064,
0.1451295614,
0.3589618802,
0.389610827,
0.2626069486,
-0.5951408744,
0.2887125313,
-0.0776977167,
-0.1011503711,
-0.1418188512,
-0.0618657172,
-0.0439789668,
-0.1890522987,
0.2088947445,
0.1767123938,
-0.221801877,
-0.0964421406,
0.4521383047,
0.2948691249,
0.1431007534,
0.1408370286,
-0.0696251988,
0.2683867812,
0.3005169928,
0.0206621289,
0.0306794792,
0.1363247782,
0.2108892947,
0.0027403699,
0.0445301272,
-0.0351566225,
0.0238793883,
0.0576749779,
-0.442152977,
0.1751865447,
0.0797956139,
-0.1001360565,
0.0160643756,
0.1829918176,
0.3118674755,
-0.2796676755,
0.1751923859,
-0.1004708335,
-0.274445653,
0.2910322845,
-0.1642244011,
0.0376680791,
-0.3985896409,
-0.0958595872,
-0.0917953625,
0.0960876197,
0.3988892436,
0.0868712142,
0.4171563685,
0.1293655187,
0.224176988,
0.1347908229,
0.275916785,
0.1784476936,
-0.0017332223,
-0.2029621452,
0.2175430655,
-0.0810274929,
-0.220953986,
-0.2160374969,
-0.2931858003,
-0.0774590299,
-0.1473876834,
0.2421828061,
-0.3560350239,
0.2010423541,
0.3800357282,
-0.1475383043,
-0.1068170518,
-0.0983295441,
0.5739682913,
-0.1218078434,
0.0830888525,
-0.084070988,
0.0201891772,
0.4858982861,
0.0717013329,
-0.2658753991,
0.2152177989,
-0.27686131,
-0.0522133037,
0.2739188969,
-0.0850306526,
-0.2639816701,
-0.2457928658,
0.1607361734,
0.1307992339,
-0.4818875492,
-0.405736059,
0.2260941118,
-0.4426643252,
-0.1356579512,
0.5243061781,
0.3977622986,
0.3573785126,
-0.108671695,
0.1821479201,
0.0534406826,
0.015882371,
-0.0299052298,
-0.129016757,
-0.194734022,
0.0986650139,
0.053240221,
-0.1217258349,
-0.0514578484,
0.2830915153,
0.0329827778,
0.3958417475,
0.0993598923,
0.0005167098,
-0.0211900827,
0.0979676917,
0.1156115383,
0.2123179436,
0.1543361843,
-0.0907528102,
0.2483416051,
-0.3218516707,
0.1370419264,
0.12543118,
-0.2333319932,
0.0656790286,
-0.1063254178,
-0.2659735084,
-0.3408952653,
0.1597789675,
0.1032045186,
-0.4180203974,
-0.2754880488,
-0.2062263489,
0.4432169795,
-0.0138521437,
-0.1089851782,
-0.2169677466,
-0.1268146187,
-0.3346157372,
0.0727747381,
-0.1406827718,
0.036524944,
0.0747133493,
-0.1410050243,
-0.164787665,
0.3544575274,
0.3195933104,
0.1061797217,
0.3022880554,
0.1927317381,
0.0328773372,
-0.1241667122,
0.1496138126,
0.0756307989,
0.1013350114,
0.0801177546,
-0.0873716101,
0.2563190758,
-0.1455116719,
-0.1093317196,
-0.1375810206,
0.1314448714,
-0.0765735433,
-0.1365863085,
-0.2957275212,
-0.1035107747,
0.610616982,
0.3171094656,
0.231996581,
0.0240162462,
-0.0837850347,
-0.0262042396,
0.1193378717,
0.112937443,
0.0377228446,
-0.1472760439,
0.2499714941,
-0.0389149114,
-0.0289269313,
0.3769489229,
0.5130671859,
0.25030756,
0.0716995969,
0.3051805794,
0.0016122821,
-0.28758201,
0.0358733945,
0.0561418235,
-0.1126170158,
0.0684216544,
-0.0435021296,
0.0493564792,
-0.1518486738,
-0.3390766978,
0.1330271661,
-0.0304418262,
-0.1809447706,
0.2108366787,
-0.0941148251,
0.2258035988,
-0.0496727079,
-0.1246386841,
-0.2088014334,
-0.4025352895,
0.2573351562,
0.0917730257,
-0.4607612193,
0.0951695666,
-0.0385734141,
0.2664901912,
-0.174666062,
0.073559694,
-0.1235507727,
-0.3214641213,
-0.0437073596,
0.0795472413,
-0.0880634338,
0.2526989281,
0.7304580212,
-0.1311330497,
-0.2009436339,
-0.3724403381,
-0.1643540263,
0.0462006144,
-0.3329816461,
0.4429240823,
0.4895552695,
0.0533523932,
-0.112060301,
-0.1844488233,
0.0840662643,
-0.3830345571,
-0.2660087347,
-0.1845017225,
0.1068546101,
-0.3374785483,
-0.3387934566,
-0.3685579002,
-0.4663521051,
-0.3014016747,
0.241975233,
-0.1762256175,
0.1574790031,
0.0984287113,
-0.4044071138,
0.0433089063,
0.2788304687,
-0.1425386965,
-0.4990940094,
-0.5869214535,
0.0771414787,
-0.2953597307,
-0.1439427286,
-0.0453417152,
0.1576923728,
-0.201232329,
0.1635487527,
-0.2414559722,
-0.745375514,
0.0937657356,
0.2354435176,
-0.0996865258,
0.0434937328,
0.377649188,
-0.0950522944,
-0.2161880136,
-0.2172878087,
0.0611052737,
0.4402587414,
-0.0263331551,
0.1234301478,
-0.021924058,
0.0885739475,
-0.0025029152,
0.5244188309,
0.23146303,
0.0415035971,
0.3437657058,
-0.191254437,
0.4083039165,
-0.0738660097,
-0.2043545097,
0.2984809279,
0.1865426004,
-0.3711796701,
0.0471346788,
-0.3468583822,
0.1800034642,
0.0091127548,
-0.0582056269,
0.2116906345,
-0.285746932,
-0.1605328619,
0.0355201215,
0.0172329303,
-0.1656160206,
0.0695134178,
0.1110110357,
-0.0095531065,
0.0143053979,
0.5648730993,
-0.0347726159,
0.1002480835,
-0.1071098894,
-0.2136799693,
-0.3458735347,
0.416901052,
-0.0235032141,
0.4492666721,
-0.3281521797,
-0.134795025,
0.2345644981,
0.0092922701,
0.4790711999,
0.1126457527,
0.1230675578,
-0.0280256309,
0.1007871628,
0.0051921215,
-0.3153509498,
-0.3267292976,
-0.0421087593,
0.1992310733,
0.0815791264,
-0.3377788961,
-0.2619325817,
-0.0124275777,
-0.2160035223,
-0.3102510571,
-0.4012080431,
-0.5420239568,
-0.2751145065,
-0.360060364,
0.2392567694,
0.1309221089,
-0.2203641534,
-0.0489161424,
0.077078037,
0.1968032867,
0.3031553328,
0.0091499677,
0.1745313406,
0.0539990254,
-0.1638088673,
0.0084144222,
0.3750194013,
0.2809550166,
-0.0584355555,
0.294231236,
-0.172145471,
0.1452233344,
0.2947829664,
-0.1858272552,
0.0139186243,
0.2652878761,
0.0881240964,
-0.0277720839,
0.0551488027,
0.3172250986,
-0.2777989507,
-0.0882018879,
0.3498711884,
0.0299353451,
-0.0799063519,
-0.0434259996,
0.0550973266,
0.0568048023,
-0.2567833662,
0.2959795296,
0.0565920323,
-0.0883460194,
0.227580443,
0.1434839964,
0.8623197079,
0.0624686033,
0.4144766629,
0.1813770086,
0.0522222519,
0.2632081509,
0.051553905,
0.0629203916,
-0.410243243,
-0.3862265348,
-0.2433965355,
-0.1412844062,
0.2726684213,
-0.3026340306,
-0.4003546536,
0.3024200499,
0.0394352563,
-0.207343787,
-0.4099215865,
0.329855144,
-0.2978416085,
-0.0898289308,
0.2729326785,
0.1163661331,
0.0611588135,
0.3924242258,
-0.0717786998,
-0.1201848611,
-0.032048326,
-0.3505805731,
-0.3782681525,
-0.2039821893,
0.0113130305,
0.3753530383,
0.0356372818,
-0.086197719,
-0.1979114264,
-0.0643184483,
-0.0133303348,
0.2368094325,
-0.1093845218,
0.222795561,
-0.0976635516,
0.023958033,
0.1493278295,
-0.3480619788,
0.3557846248,
-0.0891308635,
-0.4809230864,
-0.0553523563,
0.0374369919,
-0.2613352835,
-0.0033929534,
0.28137514,
0.3861354589,
0.0169834811,
0.0992798731,
-0.1376867443,
-0.3311130702,
-0.0852763504,
0.1279796362,
0.1529994756,
-0.2878355682,
0.2079716474,
-0.158104822,
-0.3879675567,
0.1795874387,
0.1025425866,
-0.0463120639,
-0.031726867,
0.2740697861,
-0.2099539042,
-0.0563412569,
-0.2196567506,
0.0789946094,
-0.0370825268,
0.032997679,
0.0156208612,
0.1447078288,
-0.2545281947,
0.0552964248,
0.1285775304,
0.3506358862,
0.2885817885,
-0.0284400135,
-0.4474082887,
-0.3208756149,
0.0160211232,
-0.450933069,
0.4844260812,
0.221130982,
-0.0322232321,
0.1585769355,
0.156770736,
-0.3978054523,
0.0319295302,
-0.4641882479,
-0.1248668358,
0.3408113122,
-0.0752542615,
0.0603976436,
-0.0641893521,
0.0906215906,
0.1347997189,
-0.5935949683,
-0.186376214,
-0.3097166419,
0.1394763887,
-0.1753059626,
-0.0386484005,
0.0729879364,
-0.4741796851,
-0.0042618518,
0.0098956795,
0.214598313,
0.4166865945,
-0.1314972639,
0.0742178783,
0.1948979944,
0.2095610797,
-0.0010700405,
0.2277536392,
0.1270662695,
-0.1274960339,
-0.1741638184,
0.0659340322,
-0.3609361947,
-0.1272587329,
-0.1918004751,
0.2360036224,
0.4632055759,
0.2804303169,
-0.1466748863,
0.326567173,
-0.1062844545,
-0.2187989503,
0.4266740084,
0.3294383883,
-0.2642708421,
-0.2751123905,
0.0969029292,
0.2293528914,
-0.2391540706,
-0.0873809084,
0.2002389282,
0.2963562012,
0.1713218689,
0.289986372,
0.3527079225,
0.0661031455,
-0.2116890997,
-0.0075863502,
0.3411162496,
-0.181712985,
0.157734111,
-0.1876579821,
-0.1835858822,
0.2591577172,
0.3726927936,
-0.1158643737,
0.302506119,
-0.0012335912,
-0.3071971834,
0.385319978,
0.4048245549,
0.0581487268,
0.1763492972,
0.0141605753,
0.1094287038,
-0.1097694114,
-0.0881033465,
0.1642715484,
0.1753254235,
0.5363360643,
-0.5529487729,
-0.0152975237,
-0.2336349487,
0.3381835222,
-0.1672064662,
-0.1116960123,
0.2486127466,
-0.081846267,
-0.1377280802,
-0.1703465879,
0.2336868644,
0.0222308356,
0.3978541195,
0.223487556,
0.1507522911,
-0.1550404876,
-0.1522815228,
0.094467856,
0.101366207,
-0.4949470758,
-0.1825047135,
0.3110488951,
-0.119664453,
0.4652063251,
0.337626189,
0.3382671773,
0.1054530665,
-0.146522969,
0.0138810845,
0.076686725,
0.1530277878,
-0.1337037683,
0.1422639787,
-0.0048859222,
-0.2454776466,
0.1626683027,
0.171486631,
-0.1359951645,
0.0845951065,
0.1394142807,
-0.0188519191,
-0.0488449782,
0.4834088087,
0.0646333098,
0.0362902097,
0.0416612364,
0.3051945865,
-0.6858344674,
0.1099763289,
0.0944083482,
0.1007442698,
0.1709932238,
-0.2672556937,
0.1259394139,
-0.0470306911,
0.4904878736,
0.2188588381,
0.1342142224,
-0.3599073291,
0.2000600696,
-0.6501298547,
0.5871245265,
0.0781984702,
-0.5412564278,
-0.0465625226,
0.0264715515,
0.0851541907,
0.0336237773,
0.3250843287,
0.3061598837,
-0.1271297783,
0.3344302773,
-0.3145225644,
0.1504699737,
0.0444560535,
-0.1469235867,
0.1582152992,
-0.2173132002,
0.1303638518,
0.0323743485,
0.0812971815,
0.0324244164,
0.0689333603,
0.290460825,
-0.0080099879,
0.2579009831,
0.081911318,
0.4832308292,
-0.0748587549,
-0.0566990711,
-0.0253479276,
-0.0559691042,
-0.1712684929,
-0.3650260568,
0.2243226469,
-0.0869930014,
-0.1901640445,
-0.1799831539,
-0.3332695067,
0.2587374151,
-0.2860458791,
-0.0420756526,
-0.3193356693,
0.127897352,
0.0068237227,
0.1146000028,
0.0743902996,
0.3767513037,
0.2553517818,
0.0016882784,
-0.0977998674,
-0.0905388966,
0.4677691758,
-0.3282391429,
-0.1754852384,
-0.3249821067,
0.3709001839,
0.0376721285,
0.1435144842,
-0.4403665066,
0.0035192189,
0.0317308307,
-0.0757506564,
-0.3692753613,
0.1333638877,
-0.1457455605,
-0.1425710022,
-0.1155921966,
0.1194951087,
0.103746891,
-0.4305738509,
0.1731839925,
-0.197710678
]
|
https://github.com/huggingface/datasets/issues/1954 | add a new column | Hi
not sure how change the lable after creation, but this is an issue not dataset request. thanks | Hi
I'd need to add a new column to the dataset, I was wondering how this can be done? thanks
@lhoestq | 18 | add a new column
Hi
I'd need to add a new column to the dataset, I was wondering how this can be done? thanks
@lhoestq
Hi
not sure how change the lable after creation, but this is an issue not dataset request. thanks | [
-0.2342519164,
-0.0549113378,
-0.1812906265,
-0.0524511486,
0.0165326912,
0.0354574844,
0.3398597538,
-0.0366016701,
0.0798498094,
0.1445067525,
0.0107777975,
0.1978343278,
0.0306360573,
0.3719206154,
0.1402909309,
0.1070636064,
-0.2483592629,
0.4518116713,
-0.1740529388,
-0.0729186162,
-0.3456432223,
-0.0778269544,
0.1791184694,
-0.2770397067,
-0.32051301,
-0.2202870846,
0.0320803449,
0.0979111642,
-0.3490824401,
-0.407387197,
-0.0071714367,
0.3199303746,
-0.0588899031,
0.1259932518,
-0.0001025004,
-0.1651872545,
-0.0742464662,
-0.1672582924,
-0.2324925214,
0.1484255046,
-0.2225053608,
-0.1204708517,
-0.0649787486,
-0.2121858299,
-0.4337715209,
0.0666612908,
-0.090705201,
-0.4884951115,
-0.0028184364,
0.3639039695,
0.3648744524,
0.1379201859,
-0.1298268884,
-0.3510279357,
0.275146693,
0.0080141025,
-0.1131889224,
0.1160290539,
0.1404922754,
-0.0684451014,
-0.1430791765,
0.0452408828,
0.500962913,
0.1005611643,
0.0736441761,
0.0076347748,
-0.0122204591,
-0.0688311309,
0.2871034145,
0.2532127798,
0.5526143312,
-0.1473832428,
-0.2127428651,
0.0277347565,
0.2715099156,
-0.3950328827,
-0.102369003,
0.0229897033,
0.1035378426,
0.0007530819,
0.3265816271,
-0.3593197763,
-0.3072425425,
0.1033373177,
-0.1509903073,
0.3630670607,
-0.0937534869,
-0.0232561566,
-0.1438336223,
-0.0457356647,
0.0405790061,
0.5270216465,
-0.0486874841,
0.086893186,
-0.1699163914,
-0.1534494609,
0.1258375347,
0.0771981478,
-0.0013113375,
0.0071967957,
0.0130608715,
-0.1483258009,
-0.101810351,
-0.049140662,
-0.0985416323,
-0.2586114407,
0.2241180688,
0.0814239085,
0.0712921694,
-0.1250397116,
-0.1409029514,
-0.338712275,
0.0904772133,
-0.0591128096,
0.0375071988,
-0.0255632438,
0.1908127218,
-0.1325506568,
-0.1077480614,
-0.0947955027,
0.1682733744,
-0.0805520564,
0.0298453551,
0.4504487813,
0.1969480217,
-0.0125308698,
-0.0428344421,
0.1681356579,
0.0000444408,
-0.3650208712,
-0.2425489724,
0.0406484231,
0.0234602261,
0.0446031392,
0.0642388985,
0.1984074861,
0.3595163226,
0.0659382343,
-0.0261974446,
0.1453186274,
0.173049137,
-0.039658349,
0.259041816,
0.1630304158,
-0.0337747112,
0.0389915444,
-0.0643852651,
-0.0070230281,
0.0199396648,
0.0474149026,
0.0771662742,
-0.0852074623,
-0.6027117968,
0.2963128984,
0.3269847631,
-0.2982279956,
0.1507370621,
0.1876556128,
-0.1407328695,
-0.2708616853,
0.0805227458,
0.1842940152,
-0.1445278078,
-0.1888032556,
-0.0908173174,
-0.0513374768,
-0.3442673683,
-0.0079605235,
-0.2085409909,
-0.1071882918,
0.141275689,
-0.1655450314,
-0.0701742694,
0.171363607,
-0.0367520973,
0.0876223147,
0.5381996036,
-0.0310596842,
-0.3604514897,
-0.2498098314,
-0.1576158702,
-0.3682189286,
0.2996692955,
0.5366460085,
-0.0117447702,
0.0206746832,
-0.2044988275,
0.024742458,
-0.2616029978,
-0.0983521417,
-0.1163443699,
-0.0286873318,
0.1365666538,
0.0614789575,
0.1248016581,
0.1944217384,
0.3542415798,
0.1688765585,
0.1422251314,
-0.2761054933,
-0.0772149563,
0.1145116538,
0.5605573654,
0.004230184,
-0.0603505857,
-0.0865191072,
-0.3809379637,
-0.1319014877,
-0.2419221252,
0.0342392735,
0.2410074323,
-0.3780499399,
-0.3833189309,
-0.1641272753,
-0.0116928117,
-0.0470615141,
0.2636747062,
0.1956170946,
-0.0568315387,
-0.2675726712,
-0.0989280641,
0.1625025868,
-0.0958889499,
-0.1135600284,
-0.2682310641,
0.0426567309,
-0.0947997123,
-0.1658968478,
0.2144949287,
0.180332467,
0.2492695302,
0.1912118942,
-0.0645208284,
0.18310377,
-0.0789257735,
0.2383157015,
0.2969737351,
0.1007827297,
0.0447557196,
0.1680231243,
0.0345776491,
-0.2406420708,
0.0310393516,
0.269200027,
-0.2842738628,
0.1433784068,
0.0153188268,
-0.1771541834,
0.0303520113,
-0.1171382964,
0.2075391561,
-0.3155144155,
0.1554967016,
-0.5195199847,
0.0813988969,
0.0396896489,
-0.0432147905,
-0.0339907669,
-0.3423887193,
0.2929728329,
0.1848215461,
0.1485245824,
0.2735671103,
-0.024573097,
-0.1060428247,
-0.0345942453,
-0.028358249,
0.3750407398,
0.1763317436,
0.3945907354,
0.0966876,
-0.0656398311,
-0.1075382978,
-0.2565466464,
-0.0951365456,
-0.3713628948,
0.0835187212,
0.2370495349,
0.3434161544,
-0.3087707758,
-0.4307763875,
0.097355336,
0.0629802346,
0.3789815903,
-0.2094773352,
-0.2184333652,
-0.0958884954,
0.0126109524,
0.0270038247,
0.0428648368,
0.1422491819,
-0.2052131146,
0.2989899814,
0.275551796,
-0.086921595,
-0.0169357881,
-0.2405190319,
0.3863029778,
0.151525721,
0.27850914,
-0.0031324711,
-0.350625366,
0.1799201369,
0.289672941,
-0.2606052756,
0.1840181202,
0.8060860634,
-0.1483343989,
0.2207278013,
-0.4004446566,
-0.3926119208,
-0.0963894278,
0.2622623146,
0.3963685334,
0.1159332842,
0.2579712868,
-0.0414653234,
-0.1163627952,
0.0850421414,
-0.118388474,
-0.1783897281,
-0.2418839633,
-0.2830156088,
0.3030081093,
0.0470835567,
-0.6182642579,
0.1425398737,
-0.2114256918,
0.0926958174,
0.0451595038,
0.0243388489,
-0.2476560771,
-0.1087787971,
-0.0807372257,
-0.1766065508,
0.0192313083,
-0.578874886,
-0.3256312907,
0.200262785,
-0.4118051827,
-0.2977395058,
-0.1887419075,
-0.1371979713,
0.3828651011,
-0.1759368181,
-0.0962098092,
-0.25266698,
-0.1949560046,
0.2247781754,
-0.1720817536,
0.0294295102,
0.5308919549,
-0.1651631594,
-0.2386846393,
-0.2381107062,
-0.1847110391,
0.1396242827,
0.2987616956,
0.1010990143,
-0.2928362489,
0.309417665,
-0.2106474191,
0.1787434965,
-0.2057983428,
-0.2192951739,
0.4171490967,
-0.4435091317,
0.4400139451,
0.006204138,
-0.2436643839,
0.0464620516,
0.3540068567,
0.2039541304,
0.1661486179,
0.0458549447,
0.0117861293,
0.100875929,
-0.0214097183,
-0.3324843347,
-0.1376765668,
0.0581159927,
-0.1252359897,
0.2881027758,
0.00942236,
-0.2280372232,
-0.4572381377,
-0.1159293205,
-0.1513103545,
-0.0643424466,
0.0590971559,
-0.3631083369,
-0.4082352221,
-0.0058826879,
-0.3175399005,
0.1657297015,
0.0785018429,
0.2407679111,
-0.151601702,
0.0132911848,
0.0675242916,
-0.0793042704,
0.4309130609,
-0.4250860214,
-0.0646547899,
0.1295695454,
0.1728390455,
-0.2631371319,
0.31786111,
-0.2250890583,
0.2126710564,
0.0132098459,
0.3453510702,
-0.0616825521,
0.0251383372,
0.4632738531,
0.2184384465,
-0.3159709275,
-0.2171533704,
-0.1842075884,
-0.2916347682,
-0.2276747376,
0.0814900175,
-0.087078616,
0.1912451982,
0.3089253306,
-0.1160626188,
-0.1407398134,
-0.2207106203,
0.1488068551,
0.28513062,
0.0713688582,
0.3882253766,
0.1160019189,
-0.107992284,
0.0300682578,
0.1671795696,
0.2251332998,
-0.2639663219,
0.1820215285,
0.0570058972,
-0.3748994172,
0.4564009309,
0.0895122662,
0.15828076,
0.4401010275,
-0.1284201592,
0.0947441831,
-0.1609491855,
0.2963096797,
0.3025244772,
-0.1867973357,
-0.020495275,
-0.2033823282,
0.3991322219,
-0.0663109869,
-0.2036618292,
0.2702279687,
0.2597519755,
-0.1118682399,
0.1192702428,
0.1386225224,
0.8258498907,
-0.1651406288,
-0.1446482688,
-0.0164528862,
-0.4330642223,
0.2019695789,
-0.1218136773,
0.14980115,
-0.3629226983,
-0.1199739054,
0.0581464842,
0.0594906174,
0.2272734642,
0.0732950792,
-0.0408145003,
-0.0277749822,
-0.2128304541,
-0.1205978692,
-0.0634453669,
0.3888410628,
-0.1706169695,
-0.0385124348,
0.0300870053,
0.2427951247,
0.0967733562,
-0.3202815652,
-0.0654102489,
0.1378673613,
-0.0022842379,
0.0278224777,
-0.0952258706,
-0.3391868174,
-0.0943233892,
-0.1221595332,
-0.0982921422,
-0.3562055826,
-0.0801300183,
0.1126286983,
0.4637309909,
0.22258991,
-0.1460876018,
0.3929461241,
-0.0290303081,
-0.010684805,
-0.0356687866,
0.1002593562,
-0.0205147192,
-0.2906255424,
-0.3704017103,
0.0385579243,
0.1290341169,
-0.4437743425,
-0.0630924255,
-0.0641660169,
0.1369272172,
-0.5037250519,
-0.097079888,
0.199607119,
0.1768921018,
-0.4096497893,
0.260400176,
0.2085071355,
-0.0506003611,
0.1573242545,
0.2987823486,
-0.1548347622,
-0.1539895087,
0.1537163109,
0.2887460291,
0.3733949959,
0.2711697519,
-0.1032876372,
-0.0903465897,
-0.5066464543,
0.1425129175,
0.3526087403,
-0.4496010542,
0.1566506028,
0.2619932592,
-0.1789030582,
0.1585230082,
0.2552877367,
-0.1255611777,
0.2080291957,
-0.076453574,
0.019324325,
-0.1772871464,
-0.1674601138,
-0.1012036502,
0.3335438967,
-0.0086052641,
0.1660339087,
-0.198914662,
0.2513759136,
-0.4863963127,
0.0772577077,
-0.2586611807,
0.3740481138,
0.2822451293,
0.0298458766,
0.0526983999,
-0.2013111711,
0.3311570883,
-0.1693149209,
0.0260725748,
-0.3804882467,
-0.1417731643,
0.0272810701,
-0.0407106765,
0.2253506482,
-0.1291324645,
-0.0980430543,
-0.0999184549,
0.1576021016,
0.0955435038,
-0.0132001592,
0.1009494513,
0.2714954615,
0.0844439939,
-0.0818278044,
0.0909769088,
0.060751915,
-0.0926823393,
-0.0806737319,
-0.2555574477,
0.1211445406,
-0.0813707113,
-0.0933391526,
0.2188300639,
0.07024391,
0.1460736543,
0.0843618512,
-0.0059983884,
0.0182249025,
0.1019705683,
0.3345040381,
-0.0408115871,
-0.1155473962,
0.0686179549,
0.1410688013,
0.2462551892,
0.411970526,
-0.5275210738,
0.1997170001,
0.1063261554,
-0.2075493932,
0.4894814789,
0.2371237725,
0.0950019285,
0.1738279015,
0.0527152382,
0.0670532137,
-0.1209324449,
-0.1525201052,
-0.0445638075,
0.2598795593,
0.0038234703,
-0.1028245911,
0.4838429987,
0.1744034588,
0.0401195325,
0.2454653382,
-0.0153558748,
0.3443306684,
0.1854997128,
-0.0901881903,
0.1924192309,
0.0522948988,
0.3868966103,
0.1154328138,
0.1420224458,
0.00334694,
0.2396483719,
0.3484845161,
0.0982984528,
-0.1710908115,
-0.0935465768,
0.1353798062,
-0.1843031645,
-0.2212317586,
-0.3129012585,
-0.1616816223,
-0.0202541389,
-0.1022179648,
-0.0152745331,
0.0196007099,
0.0218563806,
-0.1224045232,
0.053797666,
-0.1761559546,
0.2304534316,
-0.0224751756,
0.0916210562,
0.0089872526,
0.1721443832,
0.1660162657,
0.0933440998,
0.3902437389,
0.5117958188,
0.0263725221,
-0.1082324833,
0.1417187154,
0.3036315739,
-0.0487566404,
-0.3072278798,
-0.0955745205,
-0.1135286614,
0.2628572285,
0.2950417995,
0.0611838587,
0.3272845149,
-0.3016421199,
0.257512033,
0.0694977939,
0.0337028578,
-0.085959807,
0.0288313683,
0.1409156919,
-0.2790928185,
-0.2350943536,
-0.0579072647,
-0.1712060571,
0.0085800635,
-0.0225509182,
-0.3474242389,
0.0604504533,
-0.1142213717,
0.1349506378,
0.2431200445,
0.3177868724,
-0.036428839,
-0.075441137,
0.0554303676,
-0.3573131561,
-0.4765751958,
0.1471113712,
-0.119204849,
-0.3870982826,
-0.2098786384,
0.1780356914,
0.186948359,
0.0780627429,
0.5429639816,
-0.0980801061,
-0.0792968646,
0.0778160989,
-0.2451912314,
-0.0572219044,
0.2500937283,
0.0704836175,
-0.0130245807,
-0.0930494815,
0.1171161458,
0.0759093836,
0.2199332267,
-0.0563217998,
-0.0838340819,
0.0421148203,
-0.0226468313,
0.3171388805,
0.0366460532,
0.3963230252,
-0.3592708707,
0.0693522543,
-0.1080047563,
-0.1451852769,
-0.2400187105,
-0.0097660078,
0.4331318438,
0.3607580364,
-0.026503332,
-0.1312753856,
0.0754577518,
0.2446971387,
-0.0946356654,
0.1830009073,
-0.0927542299,
0.540756166,
0.2331819236,
-0.1764007062,
-0.0008145508,
-0.0152571602,
0.0184417274,
0.0915622339,
-0.0991520137,
-0.4116054773,
0.3260044456,
-0.0473125838,
-0.1467532814,
-0.1849722713,
0.2483227104,
0.3317495286,
-0.0286459737,
-0.3332259357,
0.2292400897,
0.2574436665,
0.0949164852,
0.1930335909,
0.447411418,
0.0441588797,
0.1114350706,
-0.07227429,
0.2434656322,
0.1745958328,
-0.1794505566,
-0.1646866947,
-0.2129771709
]
|
https://github.com/huggingface/datasets/issues/1954 | add a new column | Hi ! Currently you have to use `map` . You can see an example of how to do it in this comment: https://github.com/huggingface/datasets/issues/853#issuecomment-727872188
In the future we'll add support for a more native way of adding a new column ;) | Hi
I'd need to add a new column to the dataset, I was wondering how this can be done? thanks
@lhoestq | 40 | add a new column
Hi
I'd need to add a new column to the dataset, I was wondering how this can be done? thanks
@lhoestq
Hi ! Currently you have to use `map` . You can see an example of how to do it in this comment: https://github.com/huggingface/datasets/issues/853#issuecomment-727872188
In the future we'll add support for a more native way of adding a new column ;) | [
-0.2675051689,
-0.326751709,
-0.2293750793,
-0.0306970775,
0.0712796375,
0.1932588071,
0.2296765298,
0.0986113846,
0.2282395363,
0.1450355947,
-0.1557606608,
0.1634853333,
0.0027358972,
0.5119233727,
0.2227694988,
-0.1781964004,
-0.0625529289,
0.198812902,
-0.0534241237,
0.0027413298,
-0.349562794,
0.0484529436,
0.2379431427,
-0.1702799946,
-0.2531000674,
-0.2114896327,
-0.0195248127,
0.0866774097,
-0.2067594975,
-0.3567589819,
0.0423741899,
0.2098609358,
-0.074900277,
0.1859728545,
-0.0000973422,
-0.0641718507,
-0.0359072648,
-0.1346301883,
-0.1033531055,
-0.0347683989,
-0.2414606214,
-0.1699302197,
-0.0692437217,
-0.0861014724,
-0.4185828567,
-0.1042871699,
-0.0217462853,
-0.1531260461,
0.1256464422,
0.1134684309,
0.4063778222,
0.2553828359,
-0.0764757246,
-0.3638369441,
0.1776874363,
0.1461546868,
-0.2223311663,
0.0608459115,
0.0292490199,
-0.0947452188,
-0.0867011249,
0.2065435052,
0.374355644,
0.1480083168,
0.1906538606,
0.2405008227,
-0.1616062075,
0.0865649357,
0.1893064678,
0.3273336887,
0.4445174038,
-0.1060116217,
-0.3236677945,
-0.2550575733,
0.0308137927,
-0.3803777099,
-0.0968918353,
-0.0229369141,
0.095169127,
0.1734920591,
0.0142116379,
-0.1790037304,
-0.1423598826,
0.1836369932,
-0.2275917977,
0.2113583833,
-0.266908437,
-0.0052920044,
0.1706732512,
-0.0678176731,
-0.2897093296,
0.3743719459,
-0.0731745958,
0.1141526103,
-0.1223931387,
-0.1932972968,
0.1971587986,
0.191317901,
0.2126957774,
0.0485498272,
0.0913344324,
-0.0541548952,
-0.1107605472,
0.1634423733,
0.0828428268,
-0.1297961324,
0.2090186775,
-0.0888700038,
0.222517103,
0.0488695279,
0.082548514,
-0.3772423565,
0.2139921933,
-0.0154408375,
-0.0938183293,
-0.194900617,
0.2368553579,
-0.1049163863,
-0.1222468317,
-0.1332407743,
0.2561585605,
-0.0918195397,
0.0656535402,
0.4747041166,
0.0947092399,
-0.1584171802,
-0.045620583,
0.1981342882,
0.0805895999,
-0.1449048519,
-0.3359633088,
-0.014934442,
0.0481396765,
0.1808350533,
0.0345314927,
-0.0946614295,
0.4725376964,
-0.0024812531,
-0.0302329548,
0.1297016889,
0.0783942565,
-0.0043350463,
0.1934851259,
0.1643795073,
0.0044706319,
-0.0576050691,
0.0403549634,
-0.1187349036,
-0.1352623552,
-0.0327267461,
-0.0106868343,
-0.1250884831,
-0.3687200844,
0.3041079044,
0.1041085348,
-0.2859868705,
-0.098782979,
0.4664133489,
-0.0598141849,
-0.248629272,
0.0581968985,
0.3222053945,
-0.340573281,
-0.0693768263,
0.0408710539,
-0.0870443434,
-0.2525866926,
-0.2556756437,
-0.0349224806,
-0.2431700677,
-0.1837902814,
0.0473113358,
-0.0754371956,
0.0797241628,
-0.0735879615,
0.227775991,
0.2581998706,
0.1087667346,
-0.2271644473,
-0.1417522579,
-0.2780780792,
-0.0971001461,
0.1476490945,
0.3498809934,
0.1436209977,
0.0415607952,
-0.000869489,
0.0429342836,
-0.1848500222,
0.012602374,
0.0297190361,
-0.0185099766,
0.022534335,
0.102705799,
-0.0757717714,
0.1558427811,
0.317691952,
0.0230159145,
0.1711735874,
-0.1810259819,
-0.0701374561,
0.2003707886,
0.4800208807,
0.0673371553,
-0.1219241545,
-0.2546574473,
-0.528920114,
-0.0148892067,
0.0389765389,
-0.0887866393,
0.1192279533,
-0.3768488467,
-0.38316679,
-0.0615021437,
-0.1084754318,
-0.0569791235,
0.290710777,
0.1430886686,
0.0679391995,
-0.1913000941,
-0.1594551057,
0.1520786136,
-0.0369495451,
0.0091193728,
-0.2650410831,
0.1284197271,
0.017363444,
-0.1359552443,
0.0963119566,
0.1147401407,
0.1042971462,
0.0309454724,
0.1269075572,
0.2909141183,
-0.1034536138,
0.335962534,
0.283254981,
0.1434999704,
0.1118825823,
0.0508647896,
0.0449771732,
-0.1548962295,
0.0173944943,
0.0894270986,
-0.4237241149,
0.2668480277,
0.1428693831,
-0.0632411167,
-0.0419422537,
-0.0038181811,
0.2277863175,
-0.174127385,
0.0426246151,
-0.4620882571,
0.0182929263,
-0.0694317073,
0.089877151,
-0.026267821,
-0.4064919949,
0.2828010321,
0.337998718,
0.033987958,
0.0409408472,
0.1030982137,
-0.2593681812,
0.0518028848,
0.1649904698,
0.2050793022,
0.2228689939,
0.4660227597,
0.259660095,
-0.0010429056,
-0.1270842254,
-0.1808649749,
-0.0776133686,
-0.2090058923,
-0.0364619493,
0.1165697649,
0.2806749344,
-0.3271844983,
-0.5827215314,
0.0823419765,
-0.0810481086,
0.1986764073,
-0.1886476278,
-0.2168993354,
-0.0906993747,
0.0099924887,
-0.0009748528,
-0.2157726884,
-0.1718834341,
-0.2708531916,
0.2800835371,
0.3527167439,
-0.2082553357,
0.0128020998,
-0.0188147817,
0.2867796421,
0.040882688,
0.1994550079,
-0.0749722123,
-0.2418556213,
0.1457322687,
0.3013759255,
-0.2270243764,
0.0597374849,
0.6364294291,
-0.1969314069,
0.1622605324,
-0.401401639,
-0.4579341114,
0.045649711,
-0.0933279693,
0.2280400693,
0.1930570155,
0.4515182972,
-0.1139106452,
-0.1788261533,
0.2495382577,
-0.0848385617,
-0.3495543301,
-0.2163496315,
-0.1054066494,
0.1298490614,
-0.0546004511,
-0.3900424838,
0.0308279693,
-0.3032820225,
0.5029956102,
-0.0068599647,
0.0459585562,
0.0246300045,
0.0617438667,
0.06167138,
-0.3267001808,
-0.0044152574,
-0.5509765744,
-0.3817510605,
0.3346666694,
-0.4371896982,
-0.2686749697,
-0.0591447987,
-0.0356202982,
0.1367813647,
-0.1566548347,
-0.0953571126,
-0.3855732977,
-0.1848873049,
0.2939255834,
-0.0176991541,
0.1278983206,
0.4841797054,
-0.1433976591,
-0.2456700355,
-0.2272547334,
-0.3126324117,
0.1525457203,
0.3414380252,
0.1171691567,
-0.2383914888,
0.2780505121,
-0.0030251965,
0.294416666,
-0.0667821094,
-0.1000748053,
0.3630546927,
-0.4525366127,
0.4308472574,
-0.0333025977,
-0.2724324167,
0.1023562774,
0.170372352,
0.1682208329,
0.3327379227,
0.243400231,
0.0377826132,
0.1129042655,
-0.0568235219,
-0.1521762758,
-0.1492476016,
0.0324966945,
0.19751513,
0.1152206957,
-0.0478091165,
-0.2434741855,
-0.4107986391,
-0.0237177629,
0.0472183339,
0.1210916862,
0.0176356882,
-0.1858105808,
-0.4173742235,
0.0242549498,
-0.4365701079,
0.2714167833,
0.0817384869,
-0.1642328799,
-0.2187954783,
-0.1694922894,
-0.0801293775,
0.0094459122,
0.5412452221,
-0.1944125146,
-0.1243831441,
-0.1509484351,
-0.1719129235,
-0.2147437483,
0.3027730584,
-0.1361382753,
0.0351206288,
0.0809628889,
0.3884946108,
-0.1651664972,
-0.0014950018,
0.4199510813,
0.2146370113,
-0.2711300552,
-0.2036134899,
-0.2595190704,
-0.2660928965,
-0.2679539025,
0.1394278258,
0.0362482406,
0.0924401432,
0.4106518924,
-0.290556103,
0.0161659736,
-0.1776148975,
0.1379461139,
0.4061158597,
0.1916995496,
0.4186705053,
-0.0198622104,
0.2423339635,
0.1439826936,
0.1078943163,
0.3881812394,
-0.2044387311,
-0.1244416684,
-0.0786236152,
-0.2945459485,
0.5106817484,
0.1328925043,
0.0991695002,
0.332524538,
0.0147335213,
0.1614186913,
-0.2695332766,
0.3139100373,
0.1731737405,
0.1405241787,
0.0327329524,
-0.1346594393,
0.4818720222,
-0.0100158816,
-0.1039174274,
0.2979792655,
0.3700315058,
-0.2019833177,
0.0462890379,
-0.0542660952,
0.7508453727,
-0.167371273,
-0.0357274413,
0.0385228805,
-0.1774644107,
0.2226018757,
-0.1097049117,
0.115354687,
-0.1414429843,
-0.3266514838,
-0.0257295873,
0.1210598722,
0.2492420673,
0.073287487,
-0.0535688959,
0.0528165065,
0.0574186966,
0.1537074447,
-0.1922418773,
0.070462428,
-0.0091988388,
-0.2924911082,
-0.2694927752,
0.3007311225,
-0.0104214447,
-0.0957231522,
-0.0762967914,
0.1155963764,
0.019511003,
-0.2082200348,
-0.1271170974,
-0.3215970397,
-0.0585807338,
-0.1984930784,
0.1279904991,
-0.2477899343,
-0.2515175045,
0.1707945764,
0.4153109789,
0.0573319718,
-0.2216246575,
0.2055684477,
-0.2225227505,
0.0856782049,
-0.2475018054,
0.0399665795,
0.1094514132,
-0.177958563,
-0.2346381694,
-0.0101335375,
0.1052416563,
-0.4131992757,
-0.0338255875,
0.0321346112,
-0.2425580919,
-0.4690617025,
-0.10522037,
0.079823941,
0.1773301959,
-0.3729859293,
0.2656605244,
0.1998647451,
-0.1658199877,
-0.0440213419,
0.3708615303,
-0.127299428,
-0.151455462,
0.328745544,
-0.0637820289,
0.0696691796,
0.2002504319,
0.0091878343,
-0.1790070236,
-0.3545345962,
-0.0733046085,
0.4246882498,
-0.1535011828,
0.1729311943,
0.2348186821,
-0.2309895307,
-0.0066765808,
0.3626250625,
0.0038942371,
0.1581723392,
-0.0481378287,
-0.1246738583,
-0.4298343658,
0.0749492273,
0.0049052038,
0.3372640312,
-0.0327980667,
0.2446382195,
0.0093610529,
0.169631511,
-0.522657752,
-0.0651671886,
-0.2895472944,
0.2796229124,
0.3717901409,
-0.0287875887,
0.208365947,
-0.187535271,
0.3329309821,
-0.0352035947,
-0.1669901609,
-0.3831593692,
-0.2214453518,
0.0608603917,
0.0196372718,
0.16788055,
0.0087603061,
0.1811012924,
-0.0178547073,
-0.1832395345,
0.3455117345,
0.1026548445,
0.0202971827,
0.2028228641,
0.2382940948,
-0.069255352,
0.1074712351,
-0.1394743621,
0.0720090121,
-0.0467460826,
-0.1000200287,
-0.073156178,
-0.3047174513,
-0.0495124459,
0.3234214783,
0.1798161417,
0.2336518168,
-0.0063011362,
0.1078685299,
0.1271712482,
-0.0252149887,
0.32400769,
0.292945683,
-0.0965291485,
0.0720096231,
0.2770685852,
0.1118192226,
0.4103548527,
-0.4879569709,
0.1754483879,
0.3631244898,
-0.1352909654,
0.5029091835,
0.2272495776,
0.0658410937,
0.202155143,
0.0913500413,
0.0503359251,
0.0012865225,
-0.2114708722,
0.0581787378,
0.1365843415,
-0.0296762604,
-0.0812325478,
0.4753048718,
0.2625535429,
0.1824615449,
0.117997095,
-0.2786809504,
0.2116147876,
-0.0583934039,
0.0420127586,
0.1983389556,
-0.11374183,
0.352747798,
0.1639516205,
0.1773965806,
-0.0448387899,
0.196945712,
0.4129962325,
-0.0848415568,
-0.114660874,
-0.1688688546,
0.2937824726,
-0.100514777,
-0.2533901334,
-0.1573494971,
-0.149233982,
-0.0493523106,
-0.0913519561,
0.1106575206,
-0.1992682517,
0.373093158,
-0.1738867164,
0.1257231832,
-0.316414386,
0.0824534595,
-0.0605838671,
0.0700603276,
0.040452078,
0.1876727343,
0.1593517214,
-0.0599557683,
0.3969540298,
0.3891630173,
-0.0284739174,
-0.3357334435,
0.2878659964,
0.4870365858,
-0.2670853138,
-0.3102597296,
-0.1628081799,
-0.0846295506,
0.0545229875,
0.2395138144,
0.1223446727,
0.3468784988,
-0.3152140677,
0.2867631912,
-0.0915716514,
0.1400620788,
-0.1972036064,
0.0750785992,
0.0684300512,
-0.3613371849,
-0.1958659887,
-0.1309574544,
-0.2448280156,
-0.1584969312,
-0.0164300352,
-0.2886987925,
0.1080747694,
0.0048589613,
0.1596199274,
0.1991929263,
0.2223721147,
0.0157015882,
-0.1388692409,
0.0664107129,
-0.2817451954,
-0.4920036197,
0.0352382176,
-0.0334371626,
-0.2707376778,
-0.1485719085,
0.0685994402,
0.2237577885,
0.1569607258,
0.3889602721,
-0.1579191536,
0.0279866476,
-0.2279446125,
-0.2847082019,
-0.0991773009,
0.0880909339,
0.0599072166,
0.1327792406,
0.1024204865,
0.048295036,
0.100304842,
0.1921439767,
-0.2107074559,
0.1916918159,
-0.2847425938,
-0.2442822754,
0.1931994259,
-0.0159529597,
0.2518627942,
-0.3791729212,
0.1583208889,
-0.0179830585,
-0.1998200119,
-0.3959466517,
0.0735317394,
0.1107606292,
0.522479713,
-0.0584192723,
-0.2186154723,
0.0750895068,
0.209973067,
0.0515122525,
-0.0029991057,
-0.121534355,
0.5358170271,
0.0955800489,
-0.1547908038,
0.0824592113,
0.2031577677,
-0.0403053649,
0.0380425267,
-0.0200889558,
-0.4734791219,
0.4227636456,
-0.1249492317,
-0.2019234747,
0.0458443239,
0.0944092423,
0.2825829685,
-0.1984641403,
-0.5064719915,
0.1899572015,
0.221478194,
0.0548426285,
0.0497553945,
0.3494918942,
-0.1279302388,
0.0390068032,
-0.071811296,
0.4237107635,
0.2931394577,
-0.1638945639,
-0.0361733958,
-0.2457035482
]
|
https://github.com/huggingface/datasets/issues/1949 | Enable Fast Filtering using Arrow Dataset | Hi @gchhablani :)
Thanks for proposing your help !
I'll be doing a refactor of some parts related to filtering in the scope of https://github.com/huggingface/datasets/issues/1877
So I would first wait for this refactor to be done before working on the filtering. In particular because I plan to make things simpler to manipulate.
Your feedback on this refactor would also be appreciated since it also aims at making the core code more accessible (basically my goal is that no one's ever "having troubles getting started" ^^)
This will be available in a few days, I will be able to give you more details at that time if you don't mind waiting a bit ! | Hi @lhoestq,
As mentioned in Issue #1796, I would love to work on enabling fast filtering/mapping. Can you please share the expectations? It would be great if you could point me to the relevant methods/files involved. Or the docs or maybe an overview of `arrow_dataset.py`. I only ask this because I am having trouble getting started ;-;
Any help would be appreciated.
Thanks,
Gunjan | 113 | Enable Fast Filtering using Arrow Dataset
Hi @lhoestq,
As mentioned in Issue #1796, I would love to work on enabling fast filtering/mapping. Can you please share the expectations? It would be great if you could point me to the relevant methods/files involved. Or the docs or maybe an overview of `arrow_dataset.py`. I only ask this because I am having trouble getting started ;-;
Any help would be appreciated.
Thanks,
Gunjan
Hi @gchhablani :)
Thanks for proposing your help !
I'll be doing a refactor of some parts related to filtering in the scope of https://github.com/huggingface/datasets/issues/1877
So I would first wait for this refactor to be done before working on the filtering. In particular because I plan to make things simpler to manipulate.
Your feedback on this refactor would also be appreciated since it also aims at making the core code more accessible (basically my goal is that no one's ever "having troubles getting started" ^^)
This will be available in a few days, I will be able to give you more details at that time if you don't mind waiting a bit ! | [
-0.0609657317,
-0.2426417023,
-0.1764557511,
-0.0540160835,
0.0382514894,
-0.2388273627,
-0.1074124202,
0.2039252073,
0.2231047601,
-0.1845401525,
-0.2857308388,
0.4401740134,
-0.1111104339,
0.3817577064,
0.0005042026,
-0.2247795314,
-0.1491407007,
-0.1010930166,
-0.1609330475,
-0.021079015,
0.2228640616,
-0.0318535641,
-0.1598433405,
0.0115774861,
0.0991676599,
-0.0908597335,
0.4640901685,
-0.0603518523,
-0.3861955106,
-0.4257192612,
0.2159527242,
0.4622391462,
-0.2772479653,
0.3484129012,
-0.0001159239,
-0.1035580486,
0.3350032568,
0.0698487908,
-0.1678131968,
0.1456144154,
-0.2793073058,
-0.4648905098,
0.34608531,
0.0931734592,
-0.240172714,
-0.3023804426,
-0.4887273312,
-0.5661331415,
0.4217899144,
0.1795725375,
0.1517730802,
0.1879253238,
0.1759025306,
0.0575223342,
0.1689591855,
0.3293293715,
-0.2442489117,
-0.096868366,
0.7156177163,
-0.1107308269,
-0.070797801,
0.4140397906,
-0.060840793,
-0.1602592766,
0.3494176269,
-0.2657898664,
-0.1116121486,
-0.5176677108,
0.2471857369,
0.3313283026,
0.4620248973,
-0.1396793276,
-0.3891333938,
-0.2872939706,
-0.2797900438,
-0.1300301552,
0.0210037455,
-0.0488148406,
-0.195426479,
0.1936593503,
-0.1636241972,
-0.2893837094,
-0.3083901703,
0.0227007437,
0.2393592298,
0.263418287,
-0.2676101625,
-0.0376472026,
0.228045851,
-0.3477034271,
0.023902582,
-0.1890488416,
0.099835746,
0.3675713837,
-0.2276189923,
-0.0771990418,
0.1686148942,
0.4670667946,
0.3032638729,
0.1906507313,
-0.1145786643,
0.3745818138,
0.0616541803,
0.0119903255,
-0.0012413991,
0.0475071594,
-0.0682882145,
0.2453009039,
0.2181311399,
-0.0863912702,
-0.0993875861,
0.0604781955,
0.0828241333,
-0.2747676373,
-0.1462860852,
-0.3215170205,
-0.0542431958,
-0.4708522558,
-0.0017118508,
-0.1667613834,
-0.0813659951,
-0.2673493028,
0.4737507105,
0.219733417,
-0.1195388362,
-0.0570731349,
-0.2483891696,
-0.1011036411,
-0.1768928319,
-0.002942319,
0.0027064185,
-0.1255302876,
-0.1310471594,
-0.1374054402,
0.1485550255,
-0.1948986053,
-0.0996299013,
0.0176745448,
-0.0331115797,
0.2060009241,
0.2575750351,
0.0909350738,
0.3814997673,
0.3757706881,
-0.401373148,
-0.0247619543,
0.1775444448,
0.0400690585,
-0.2153910547,
-0.0036564623,
-0.2171406746,
-0.5327576995,
0.0032876173,
0.0952743962,
-0.2179054767,
-0.1383994222,
-0.1729110181,
0.6192803383,
-0.213228941,
-0.0004947346,
0.1261021942,
0.0303850342,
-0.0746980309,
-0.2937883437,
0.3139741719,
0.2567201257,
-0.490203768,
-0.0608593449,
-0.2886766791,
-0.1347985566,
0.2083832026,
0.1620912999,
-0.4143378437,
-0.2132015675,
0.1621504128,
-0.0254683793,
0.5568777323,
-0.1211440861,
-0.3929442465,
0.0143117253,
-0.0648193955,
0.3086546659,
0.0995054767,
0.0061433055,
0.2001387328,
-0.1530795693,
0.0048773969,
0.6350787878,
-0.165750131,
-0.1484430134,
-0.3304504454,
-0.0936997682,
0.0300594512,
0.0449817516,
-0.0954459161,
-0.0510532223,
0.1706355512,
-0.5012770295,
0.1360241026,
0.0712877661,
0.1759145111,
-0.0128357736,
0.4707922935,
0.2733280063,
0.3618062139,
0.2172621936,
-0.2060659528,
0.2344364673,
0.0301133972,
-0.1215243042,
-0.4391418397,
-0.057195235,
-0.0998984948,
0.1922445297,
-0.1285838187,
-0.0275910702,
0.0785060525,
-0.2916638553,
0.2678439021,
-0.1258757263,
-0.3642390966,
0.2730606794,
-0.1230476499,
0.0722341016,
-0.1536017209,
0.0954931155,
0.2370284647,
0.1554509401,
-0.0102557363,
-0.090042375,
-0.0304582864,
-0.1076328084,
-0.1002158672,
0.006186483,
0.1352831125,
0.2469384819,
0.40576455,
0.5706694126,
0.1249559224,
-0.4567499757,
0.412987709,
0.0831521675,
0.1664698422,
0.0793101266,
-0.273856312,
0.7109123468,
-0.496045202,
0.1653508544,
0.1060895026,
0.0411677808,
0.0584858879,
0.1099970043,
-0.3593266606,
0.1287241429,
0.2998859882,
-0.3171957731,
0.2916971743,
-0.0638212413,
-0.1837733686,
0.4710715115,
-0.1567674726,
0.1237306148,
-0.245021686,
0.3046878278,
0.0002447359,
-0.1571686566,
0.0060963836,
0.3676765263,
0.0476040319,
0.3104172647,
0.074371554,
-0.196602881,
0.1642753482,
0.0204417799,
0.3753751218,
0.0974474028,
0.3182748258,
-0.0777940527,
0.2552366257,
-0.0056445366,
-0.2419346869,
-0.3230294585,
-0.164876461,
0.1371055096,
-0.1429522783,
-0.0491913706,
0.1000466868,
-0.3030158877,
0.0799468756,
-0.4713405669,
-0.0805587471,
-0.0791647062,
0.1630920023,
-0.1481856853,
-0.0590705425,
0.1847272217,
-0.3384582996,
0.2940368354,
-0.0116716214,
-0.2430038452,
-0.3440414965,
-0.1907556057,
0.1860197783,
0.0658501685,
0.0843952671,
0.2708716393,
0.3436903656,
0.391938895,
0.0891069844,
-0.1109160632,
-0.6208747029,
0.037650574,
-0.0244236607,
0.3294963241,
-0.0550893992,
0.170315817,
0.0902503356,
0.0219802223,
0.2207975686,
-0.183091566,
-0.0867152736,
-0.2048918456,
-0.2004707456,
0.1766234636,
0.1549230218,
0.0479064621,
-0.4060261548,
-0.3476782143,
0.2588632107,
0.0216844436,
0.1105115116,
0.1468439251,
0.1031277478,
0.1100964397,
-0.3070745766,
0.2067844421,
0.0703001693,
-0.0732144639,
0.275093466,
-0.1457666755,
-0.2073545605,
-0.1615957916,
-0.23630777,
-0.0982560888,
0.3142912984,
-0.1593424529,
0.0102771167,
-0.4495510459,
0.283490479,
0.0864340812,
0.0271232463,
0.334581852,
0.0846132264,
0.0097950064,
-0.2262040377,
-0.2326354831,
0.0154640405,
-0.1563066244,
0.1400000602,
0.3474458754,
0.356659323,
-0.0228052177,
0.7490891218,
0.2729084194,
0.3102784157,
-0.0216604229,
0.2651238143,
-0.0104621341,
-0.0047168243,
-0.3897791803,
-0.207657516,
-0.183205381,
0.0713236481,
0.0647227243,
0.470816642,
-0.2996090353,
-0.2581761479,
-0.1289067119,
-0.2300783247,
-0.1261657774,
0.1658202857,
-0.0819253996,
0.2298604995,
0.021534685,
-0.2481346428,
-0.3278769553,
0.0212044958,
0.093988888,
0.1197003424,
0.3694303632,
-0.115592584,
-0.3070031404,
-0.0110323522,
-0.4549894333,
0.3740396798,
0.0163292885,
-0.0884352922,
-0.0765640065,
-0.1763865501,
0.1765039414,
0.0622376464,
0.4188169241,
-0.1716433913,
-0.2019243836,
0.0084139127,
-0.210238412,
-0.3476480246,
0.3733869791,
-0.1467583477,
0.1551926434,
-0.163620472,
0.1940011382,
-0.2316621989,
-0.4352599084,
0.0153542757,
0.0025668568,
0.1551007628,
0.135179162,
0.1571703702,
-0.2165167183,
-0.0974029303,
0.2244460434,
-0.0569242127,
-0.1157419384,
-0.0190442596,
0.1077828333,
0.0715732649,
0.2140100002,
-0.0328814462,
0.0305707138,
0.1167550012,
0.1374803334,
0.1845524162,
0.2986931801,
0.0114757037,
-0.0831662044,
0.498640269,
0.0181760322,
-0.1970019788,
-0.4065935612,
0.3767667413,
0.4093342125,
0.6419485211,
0.0083802566,
0.4672680497,
0.1627708524,
0.125239417,
0.0951695144,
-0.2514248788,
0.0303576794,
0.1789839119,
-0.4732811749,
-0.4895402491,
0.5944272876,
0.0797556713,
-0.2069956213,
0.6742950678,
0.4888077378,
-0.021364158,
0.2731508315,
0.0317816101,
0.938129425,
0.1353501379,
-0.2550575733,
-0.0551718511,
-0.4572876692,
0.2276631296,
0.1907259971,
0.0677271336,
-0.1280802935,
-0.0280981306,
0.0904291868,
-0.1635539532,
0.2674818337,
-0.0624956563,
-0.139267385,
-0.1324778944,
-0.0148853334,
-0.3627676368,
-0.1140648946,
0.1413877457,
0.1497002095,
-0.3225054443,
-0.4596540034,
0.1375409514,
0.0297196861,
0.2756155729,
0.1242969185,
-0.3360019028,
-0.1396520734,
0.0116647473,
-0.1610211432,
-0.2538188696,
-0.4586697817,
0.0900087804,
0.3469234109,
-0.6894706488,
0.4541457593,
-0.2189526707,
-0.0276081245,
0.0240771398,
-0.2672505379,
0.3191044033,
0.3087885678,
0.0923416913,
0.1026988477,
-0.044827342,
0.4351015389,
-0.1017231569,
-0.1091321558,
-0.1032358333,
-0.2462424636,
-0.3320295811,
-0.1245476902,
-0.1183080524,
0.4498855472,
0.0405548438,
-0.1221790537,
0.1863027811,
-0.232357353,
-0.075032495,
0.1450914443,
0.2975462973,
-0.1378027052,
0.3352734149,
0.2150521576,
-0.0183793958,
-0.0883292034,
0.1541645378,
0.011942476,
-0.2380981296,
0.1957040131,
0.4382346272,
-0.3694272935,
-0.0726268515,
-0.0423767716,
0.4137722552,
-0.3821430504,
0.0919387639,
0.0623242408,
0.000077105,
0.3333821893,
0.2797308266,
0.0447165184,
-0.1187899709,
-0.2067810297,
-0.2249266505,
-0.2354303151,
0.2200052142,
-0.2416063547,
-0.0036623853,
-0.6351539493,
-0.3681564629,
0.1231981069,
0.2730613351,
-0.3414293528,
0.1466782093,
0.178866297,
0.0029469884,
0.1062559187,
0.1206168756,
0.2502304316,
0.0875688344,
0.0558764935,
-0.1611802578,
-0.4421406388,
-0.1634099931,
0.0420132726,
0.1547055095,
-0.2273909152,
-0.3869339228,
0.1276438981,
-0.2015356123,
-0.4394990802,
-0.0248724204,
0.0112370448,
0.1066527367,
-0.0087063313,
-0.4182298183,
-0.1489042789,
-0.3942818642,
0.1087363139,
0.118557848,
0.1818754524,
-0.053211417,
0.2519567311,
0.072556667,
-0.0979095995,
-0.2889057696,
0.068379119,
0.2930141389,
0.0831350759,
-0.1490927786,
0.3740787804,
-0.3148295581,
-0.020864008,
0.0578760169,
0.4791159928,
0.1315641254,
0.0495789573,
0.2891439199,
0.2545942068,
0.1510764807,
-0.2919921279,
-0.0689227954,
0.2296598107,
0.0560886301,
0.1602948457,
0.0991425216,
0.1550761163,
0.3570215404,
0.1556580663,
0.1796746552,
0.1728065759,
-0.1825160682,
0.0343783721,
0.0758162513,
0.2442068011,
-0.2312832028,
0.5170526505,
0.4599159062,
0.1527621746,
0.2112319767,
0.0077617024,
0.3438524008,
0.1532797366,
0.3685175776,
-0.0573915504,
-0.3248311281,
0.1044098511,
0.1820530146,
-0.3671521842,
0.1109519228,
0.0719505623,
0.4479799569,
0.2105863541,
-0.0966872945,
0.1776972711,
0.2294196934,
0.1842697412,
-0.1984756291,
0.2682887614,
-0.4554391801,
-0.0567954704,
-0.0700844377,
-0.1246257499,
0.1792716384,
0.5062456131,
-0.033642251,
0.4923926592,
-0.3771257102,
0.0177506637,
0.1347044855,
0.5880486965,
0.0054554166,
0.1343385875,
-0.0756397992,
-0.0395637266,
0.0040606358,
0.1912314743,
0.2396371067,
0.0882500634,
-0.221531406,
0.1217185557,
-0.1278240681,
-0.3407157063,
0.0758995637,
0.5048359632,
0.3018205762,
-0.3505637348,
0.0060390378,
0.0432860404,
-0.1411369294,
-0.0027774833,
-0.1935842633,
-0.018446764,
-0.0177156962,
-0.0776143447,
0.0754840374,
-0.1306014508,
-0.4775841832,
-0.1475536823,
-0.1269415021,
-0.2714186907,
0.2402142584,
-0.2049628943,
-0.1174962074,
-0.0226438437,
0.0711856037,
-0.0046641659,
0.5002952814,
0.2632887065,
0.384891659,
-0.0844281465,
-0.3183429837,
-0.22902219,
-0.3159652948,
-0.1155212969,
0.1433747709,
0.2399310619,
0.0960695297,
0.307060957,
0.4269804657,
0.1642242223,
-0.3078043759,
0.2154695988,
0.1345232725,
-0.2297237813,
0.2803106904,
0.2107989937,
0.3605068326,
-0.1337548494,
0.0139038721,
0.0631634891,
-0.3434785903,
0.0417123623,
-0.11770612,
0.1252274364,
0.2732971013,
0.0424073115,
0.0395917147,
0.1712903678,
0.2689300776,
0.1354906857,
-0.0002491429,
-0.2420799732,
-0.1890339106,
-0.0756740645,
0.1631831676,
0.1734728962,
0.365272969,
0.081143029,
-0.4510498047,
-0.0391220599,
-0.0669402927,
-0.2234857231,
-0.0710235313,
0.0192720741,
-0.0203315895,
0.0477411523,
0.0642383397,
-0.2072573006,
0.2440376133,
0.1739784032,
0.0113016414,
-0.406594336,
-0.2832552195,
0.6696615219,
-0.4198069274,
-0.2190533131,
-0.1257773191,
0.0656543151,
0.3203107715,
-0.1362005323,
-0.247834757,
-0.1276456863,
0.0048934901,
-0.2941201031,
-0.0921110883,
0.3316379488,
0.0929636806,
0.0761643201,
-0.0724549368,
0.1414699554,
0.1727241427,
0.2042234838,
-0.4060376585,
0.0155453961
]
|
https://github.com/huggingface/datasets/issues/1949 | Enable Fast Filtering using Arrow Dataset | Sure! I don't mind waiting. I'll check the refactor and try to understand what you're trying to do :) | Hi @lhoestq,
As mentioned in Issue #1796, I would love to work on enabling fast filtering/mapping. Can you please share the expectations? It would be great if you could point me to the relevant methods/files involved. Or the docs or maybe an overview of `arrow_dataset.py`. I only ask this because I am having trouble getting started ;-;
Any help would be appreciated.
Thanks,
Gunjan | 19 | Enable Fast Filtering using Arrow Dataset
Hi @lhoestq,
As mentioned in Issue #1796, I would love to work on enabling fast filtering/mapping. Can you please share the expectations? It would be great if you could point me to the relevant methods/files involved. Or the docs or maybe an overview of `arrow_dataset.py`. I only ask this because I am having trouble getting started ;-;
Any help would be appreciated.
Thanks,
Gunjan
Sure! I don't mind waiting. I'll check the refactor and try to understand what you're trying to do :) | [
-0.1475262791,
-0.0889887288,
-0.2098283321,
-0.0918973088,
0.0624384992,
-0.2331447154,
-0.0470119081,
0.2564984262,
0.1409167498,
-0.1652854979,
-0.2117006183,
0.5572013855,
-0.1610823274,
0.234077096,
-0.0721702352,
-0.1115352735,
-0.163025558,
-0.033403445,
-0.1529835612,
-0.057040669,
0.1605863422,
-0.0926774144,
-0.169358477,
-0.0569528863,
0.0641344786,
-0.0823348165,
0.4899252653,
-0.1223513559,
-0.3783578575,
-0.5257979035,
0.1804880053,
0.4125717878,
-0.2424082011,
0.2819819748,
-0.0001122101,
-0.0977283046,
0.3756689727,
0.0460014455,
-0.1633183509,
0.201804921,
-0.3833836317,
-0.5163578391,
0.3023419678,
-0.0402291231,
-0.2140235007,
-0.3167769313,
-0.4475967288,
-0.6233633161,
0.3333079219,
0.2781322896,
0.197769016,
0.0779645145,
0.129611969,
0.0343554653,
0.2299923748,
0.1702784449,
-0.275714159,
-0.161130935,
0.6908950806,
-0.1348017305,
-0.1131288111,
0.4136621356,
-0.0534188598,
-0.1600667834,
0.261238277,
-0.3271079063,
0.0970114395,
-0.514010489,
0.2721893787,
0.2440672666,
0.5241883993,
-0.0686450601,
-0.2503682673,
-0.115498662,
-0.295946151,
-0.0541288145,
-0.0665445551,
-0.0593074299,
-0.1523034573,
0.1953793019,
-0.1410024464,
-0.3106040657,
-0.3834902942,
0.0470867865,
0.1975132525,
0.3864696324,
-0.1185575575,
-0.0790665597,
0.1377028823,
-0.3078045547,
0.2436962426,
-0.1139411703,
0.0077826451,
0.3805400133,
-0.2030076832,
-0.0627388954,
0.1559076756,
0.4094283581,
0.2585113943,
0.129013285,
-0.05375484,
0.3599945009,
0.1209416315,
0.0324547514,
-0.0365007222,
0.0077356421,
-0.0455787852,
0.4321997166,
0.1505798399,
-0.207145676,
-0.2090796828,
0.0376980491,
0.0157881491,
-0.2357440293,
-0.0105470428,
-0.2417123914,
-0.0886398926,
-0.4509323239,
-0.0292539056,
-0.1328237355,
-0.1488555968,
-0.2649077177,
0.3727761507,
0.2461597919,
-0.0908477008,
-0.0422479212,
-0.277698338,
-0.1313284487,
-0.2057757974,
0.0612552464,
0.0159736704,
-0.102088213,
-0.1534935832,
-0.2902714312,
0.1802893877,
-0.0157663468,
-0.1032722145,
-0.0190819521,
-0.07435105,
0.2151857913,
0.3460691273,
0.0992514491,
0.4113320112,
0.3538832366,
-0.4210656881,
-0.0184229147,
0.1159557402,
0.0654643178,
-0.2028470188,
0.1139784753,
-0.2340941727,
-0.507129848,
-0.0122120241,
0.1530790627,
-0.166732654,
-0.1694043875,
0.0689222962,
0.5219019651,
-0.1484540254,
-0.053949777,
0.1301654577,
-0.0499788299,
-0.0169825014,
-0.354963094,
0.1976926923,
0.1616175175,
-0.5772004128,
0.0571638644,
-0.3905584514,
-0.0376621708,
0.2938017845,
0.1009635478,
-0.4094146192,
-0.2548652887,
0.1447504312,
-0.0853995532,
0.6621947885,
-0.1478879601,
-0.5032476783,
0.0212398134,
-0.1166823655,
0.2362808734,
0.1395218074,
0.0708171651,
0.2376328856,
-0.1459854096,
0.0106072361,
0.5886855125,
-0.1571552306,
-0.1358808279,
-0.3851483166,
-0.0330214277,
0.0929221213,
0.0147239277,
-0.0122917788,
-0.048901882,
0.2204796225,
-0.3853614926,
0.1980081946,
0.0757928789,
0.1681530178,
-0.0461235382,
0.5657212734,
0.1406770647,
0.3982256353,
0.2781225741,
-0.0813047811,
0.2465828955,
-0.0564614013,
-0.100546442,
-0.3765895069,
-0.0060680066,
-0.1080691367,
0.1723629683,
-0.1178417802,
-0.0534228683,
0.1456341445,
-0.258621335,
0.1876116395,
-0.111932762,
-0.2767267525,
0.1864238828,
-0.1401676238,
-0.0221047904,
0.077728726,
0.0473793745,
0.1509614885,
0.1477292627,
-0.0362886116,
-0.2032632828,
-0.0335148014,
-0.0979412273,
-0.1415888816,
-0.0482923388,
0.1992948055,
0.2076706439,
0.3779622912,
0.5796355605,
0.0671273023,
-0.4598430991,
0.4000549018,
0.1309715062,
0.1228170842,
0.0600436963,
-0.2230873406,
0.6486537457,
-0.4913369417,
0.1301897466,
0.0743035302,
0.0571305044,
0.1209031567,
0.0916388258,
-0.3350031972,
0.1061153561,
0.2322735786,
-0.2754031718,
0.27043432,
-0.046651043,
-0.105330199,
0.4582202435,
-0.1308723986,
0.2124438882,
-0.2018692642,
0.2182040513,
0.0384848788,
-0.1373000592,
0.0497140214,
0.477694273,
0.0552190244,
0.3242242634,
0.1072231978,
-0.235141024,
0.0779880062,
0.0317963064,
0.4003837705,
0.033239089,
0.3895936608,
-0.0226084813,
0.2586922646,
-0.0754907429,
-0.1984191239,
-0.333596915,
-0.0943608433,
0.1433905214,
-0.0976475254,
-0.1084431186,
0.124360539,
-0.278676182,
0.0896420702,
-0.4824868739,
0.0609075613,
-0.118247591,
0.1991486102,
-0.2700443268,
-0.0258984957,
0.2583580911,
-0.4764938056,
0.2215437293,
0.0698766187,
-0.2258891314,
-0.2692490518,
-0.2329247296,
0.1807038039,
0.1188024506,
0.0096917134,
0.2544659078,
0.3076719046,
0.4234209657,
0.185579583,
-0.0607868321,
-0.5851411223,
0.0178728327,
0.0574482977,
0.2471531332,
-0.1005005091,
0.0984465182,
0.0665507838,
0.0384892039,
0.1948616505,
-0.177629143,
0.0035022162,
-0.2151725739,
-0.1799064428,
0.1618774533,
0.181491375,
-0.0074707409,
-0.4025439322,
-0.3848939836,
0.0839818716,
0.0953596607,
0.1507595032,
0.0864574388,
0.1575747132,
0.0498173349,
-0.2199696451,
0.1554562896,
0.1019882336,
0.0315357484,
0.3705922663,
-0.1950345635,
-0.18306005,
-0.1638772339,
-0.2358261496,
-0.0301395673,
0.3896688223,
-0.1173680127,
0.1325733513,
-0.3845604062,
0.265075624,
0.0392279364,
-0.0429853462,
0.2871625125,
0.1398594826,
-0.0358022898,
-0.2890632153,
-0.0778522342,
0.0468320772,
-0.1745107621,
0.1589364409,
0.2671410739,
0.2515631616,
-0.0135229044,
0.6781638265,
0.2385451347,
0.3053128421,
-0.0278056301,
0.2382787168,
-0.0804036334,
0.0050878404,
-0.3636898696,
-0.2951539159,
-0.1504164487,
-0.051560875,
0.0314597748,
0.39229092,
-0.5023053885,
-0.2534082532,
-0.107609801,
-0.1740995198,
-0.0939804316,
0.2185050845,
-0.2374885827,
0.2750199139,
0.0177652799,
-0.1958896816,
-0.3312812746,
0.0534341,
0.0893673524,
-0.0237316843,
0.3159022331,
-0.1551205367,
-0.2472106963,
-0.0224768855,
-0.3784747422,
0.3182934821,
-0.007368831,
-0.0131219504,
-0.0167229418,
-0.1759759784,
0.1313331425,
0.0682748854,
0.3394866884,
-0.2564072907,
-0.1299345791,
0.0996437222,
-0.0654613823,
-0.2634759545,
0.3052333593,
-0.1720009446,
0.2245873809,
-0.1720319837,
0.1267335713,
-0.0689733103,
-0.2498935312,
-0.0183734745,
-0.0665833056,
0.1111677587,
0.1037799865,
0.2244230211,
-0.1516264081,
-0.0731593445,
0.2071255147,
-0.0555612519,
-0.1221343055,
-0.0203144364,
0.0570162758,
0.055757761,
0.2012998462,
-0.0699209943,
-0.0022003739,
0.1236116365,
0.0978467464,
0.1559081227,
0.1842033267,
0.0010706955,
-0.0571027286,
0.4396477044,
-0.0187203437,
-0.0930866972,
-0.4215840995,
0.3052025139,
0.41747877,
0.5442765951,
0.0049809171,
0.3545247614,
0.0523049645,
0.0445028991,
0.2079080641,
-0.2735942006,
0.0200397316,
0.0816987678,
-0.4799918234,
-0.552965045,
0.5566237569,
0.044552464,
-0.2231653929,
0.6911585927,
0.2764504552,
-0.0742663294,
0.3161837161,
0.1092559174,
0.8662747145,
0.1756537557,
-0.3162349463,
-0.18965666,
-0.451664567,
0.1645270586,
0.1649384499,
0.0966425315,
-0.1555040181,
-0.0197431259,
0.1007923111,
-0.1575295776,
0.23834759,
0.0757959709,
-0.1866053194,
-0.1635291874,
-0.0692257732,
-0.4089562595,
-0.1013877615,
0.1564002335,
0.191961363,
-0.2369518876,
-0.3374944627,
0.1550123096,
0.0376731828,
0.1941006333,
0.1098065004,
-0.2859632373,
-0.1854333133,
0.0339031816,
-0.1372966319,
-0.209848851,
-0.4218374193,
0.2017422616,
0.1767585278,
-0.6858208179,
0.403275162,
-0.2590162456,
-0.1760680377,
0.0660737753,
-0.2633847892,
0.3766176701,
0.4508700669,
0.0541925356,
0.1525779814,
-0.0431578308,
0.3689368069,
-0.1130988896,
-0.1333815753,
-0.0969928503,
-0.3404677808,
-0.3378997445,
-0.2013334781,
-0.073895812,
0.5246477723,
0.1063145846,
-0.1258168817,
0.1729200482,
-0.3183771372,
-0.0435610935,
0.2024710476,
0.2792019844,
-0.1081268638,
0.4356886148,
0.2355085462,
-0.0234058071,
-0.0776551142,
0.1419812888,
0.1190922186,
-0.1750059873,
0.1872940361,
0.3225567043,
-0.3461341262,
-0.1163361222,
-0.004614518,
0.3299773037,
-0.2947356403,
0.0382046625,
0.0107052131,
0.0373413116,
0.3760465682,
0.2310848534,
0.0844662413,
-0.0195480958,
-0.3427931666,
-0.1993968785,
-0.2802717984,
0.1333852112,
-0.2288032025,
0.0179556962,
-0.6987082958,
-0.3938128054,
0.1250785589,
0.2507771254,
-0.3786207139,
0.1363943815,
0.1850525886,
0.0663277656,
-0.0127425203,
0.1230913475,
0.1247163787,
0.1519986391,
0.107539624,
-0.1399761438,
-0.369569093,
-0.1927405447,
0.1210189983,
0.1453264952,
-0.1919301897,
-0.345367372,
0.0982168317,
-0.2254564017,
-0.4630483389,
0.0646003187,
-0.1146151051,
0.0931686088,
-0.0822218657,
-0.329838872,
-0.1761527658,
-0.4999933243,
0.1140103266,
0.117586337,
0.1143504307,
-0.0884715617,
0.2574736178,
0.159389317,
-0.0012379668,
-0.2922891676,
0.0625132248,
0.2208312899,
-0.0397203192,
-0.0318804123,
0.4271561503,
-0.2684900463,
0.0735092312,
0.0119834095,
0.428999126,
0.0997654274,
-0.0149691012,
0.2136554718,
0.237022385,
0.2167619765,
-0.2880710065,
-0.1528850645,
0.2142185569,
-0.0141338008,
0.1783148646,
0.09579584,
0.1697102487,
0.2545416653,
0.1227245554,
0.1712328494,
0.1429407746,
-0.2657508552,
-0.0098017287,
0.2091665715,
0.1680660248,
-0.2541534603,
0.453797251,
0.3679918647,
0.1609521955,
0.3294355869,
0.087291576,
0.4186423719,
0.2954576313,
0.3099859357,
-0.0988232195,
-0.2240899801,
0.1348629892,
0.1933923364,
-0.3778964281,
0.189337045,
0.1609069556,
0.2772224545,
0.2905344069,
0.010082257,
0.1538791209,
0.1100173593,
0.1400523335,
-0.1770975292,
0.2699900568,
-0.4617326558,
-0.0446577631,
-0.0875974,
-0.1264298856,
0.3221155107,
0.4303366542,
-0.0477121174,
0.4564765692,
-0.2363157868,
0.1498157382,
0.0908817798,
0.5641852617,
-0.1071923971,
0.0874123871,
-0.1085969135,
-0.0462370068,
0.0002310232,
0.0986825079,
0.2250643522,
0.2264629006,
-0.1615529656,
0.0607653856,
-0.0552969053,
-0.3745654821,
-0.0088106254,
0.5079407096,
0.3447356522,
-0.3521857262,
0.0400463864,
0.0511242524,
-0.1720167994,
0.078404963,
-0.1419507414,
-0.0302250739,
0.0869737566,
-0.1116787121,
0.1773303747,
-0.2569565773,
-0.4046736956,
-0.102426216,
-0.0818588808,
-0.3288163543,
0.2311628908,
-0.2292253375,
-0.0891657099,
-0.0596247092,
0.0937024057,
-0.075682573,
0.5075572133,
0.1621737182,
0.3664550185,
-0.0580714382,
-0.3708944917,
-0.2053265721,
-0.1831038445,
-0.2422369421,
0.1734286696,
0.2796366811,
0.1507984251,
0.2834040821,
0.4105999768,
0.2246814817,
-0.2500523329,
0.1204195693,
0.2884008586,
-0.2290095538,
0.2530552745,
0.2458892763,
0.3859765232,
-0.1710739583,
-0.0497931615,
0.0808863193,
-0.3277787864,
0.1301948428,
-0.1378729641,
0.0346539989,
0.4280060232,
0.1171978787,
0.1686580926,
0.1115587875,
0.3198148608,
0.1346817464,
0.0524807461,
-0.2619464695,
-0.2163195014,
-0.0217668116,
0.1465343386,
0.2841081917,
0.3330195844,
0.1076079011,
-0.4194907546,
0.0117202364,
-0.1147374287,
-0.3243845701,
-0.0019164977,
0.0411208011,
-0.0516377315,
0.0358679369,
0.0699701533,
-0.3306316137,
0.1953561753,
0.1989714056,
-0.0378248692,
-0.4084859192,
-0.2806215286,
0.5808034539,
-0.3440422118,
-0.2982539833,
-0.0936834738,
0.0427404679,
0.1809696704,
-0.0274205841,
-0.1848164648,
-0.166557461,
0.1074379757,
-0.3471878767,
-0.0620349944,
0.2725346088,
0.1391054392,
0.1319850832,
-0.0350427702,
0.0918882564,
0.1254366785,
0.2822115123,
-0.5548897982,
-0.0043712864
]
|
https://github.com/huggingface/datasets/issues/1948 | dataset loading logger level | These warnings are showed when there's a call to `.map` to say to the user that a dataset is reloaded from the cache instead of being recomputed.
They are warnings since we want to make sure the users know that it's not recomputed. | on master I get this with `--dataset_name wmt16 --dataset_config ro-en`:
```
WARNING:datasets.arrow_dataset:Loading cached processed dataset at /home/stas/.cache/huggingface/datasets/wmt16/ro-en/1.0.0/9dc00622c30446e99c4c63d12a484ea4fb653f2f37c867d6edcec839d7eae50f/cache-2e01bead8cf42e26.arrow
WARNING:datasets.arrow_dataset:Loading cached processed dataset at /home/stas/.cache/huggingface/datasets/wmt16/ro-en/1.0.0/9dc00622c30446e99c4c63d12a484ea4fb653f2f37c867d6edcec839d7eae50f/cache-ac3bebaf4f91f776.arrow
WARNING:datasets.arrow_dataset:Loading cached processed dataset at /home/stas/.cache/huggingface/datasets/wmt16/ro-en/1.0.0/9dc00622c30446e99c4c63d12a484ea4fb653f2f37c867d6edcec839d7eae50f/cache-810c3e61259d73a9.arrow
```
why are those WARNINGs? Should be INFO, no?
warnings should only be used when a user needs to pay attention to something, this is just informative - I'd even say it should be DEBUG, but definitely not WARNING.
Thank you.
| 43 | dataset loading logger level
on master I get this with `--dataset_name wmt16 --dataset_config ro-en`:
```
WARNING:datasets.arrow_dataset:Loading cached processed dataset at /home/stas/.cache/huggingface/datasets/wmt16/ro-en/1.0.0/9dc00622c30446e99c4c63d12a484ea4fb653f2f37c867d6edcec839d7eae50f/cache-2e01bead8cf42e26.arrow
WARNING:datasets.arrow_dataset:Loading cached processed dataset at /home/stas/.cache/huggingface/datasets/wmt16/ro-en/1.0.0/9dc00622c30446e99c4c63d12a484ea4fb653f2f37c867d6edcec839d7eae50f/cache-ac3bebaf4f91f776.arrow
WARNING:datasets.arrow_dataset:Loading cached processed dataset at /home/stas/.cache/huggingface/datasets/wmt16/ro-en/1.0.0/9dc00622c30446e99c4c63d12a484ea4fb653f2f37c867d6edcec839d7eae50f/cache-810c3e61259d73a9.arrow
```
why are those WARNINGs? Should be INFO, no?
warnings should only be used when a user needs to pay attention to something, this is just informative - I'd even say it should be DEBUG, but definitely not WARNING.
Thank you.
These warnings are showed when there's a call to `.map` to say to the user that a dataset is reloaded from the cache instead of being recomputed.
They are warnings since we want to make sure the users know that it's not recomputed. | [
-0.1510904878,
-0.363607198,
-0.0170672107,
0.3475189805,
0.4003513455,
0.4622991085,
0.4706436396,
0.1432848126,
0.2523233891,
-0.0047668768,
0.0243789218,
-0.0168784354,
-0.2481700927,
-0.1975330263,
-0.3672414422,
0.1944216043,
-0.1171082929,
-0.0153789278,
-0.3518668711,
-0.0757232234,
-0.1171179935,
0.0906820893,
-0.0266270209,
0.5081021786,
-0.7216522694,
-0.0836910158,
0.1400513649,
0.118843466,
0.0099194786,
-0.7821525931,
0.2707005441,
-0.1280101091,
0.14961119,
0.0640892684,
-0.000122115,
0.2060292065,
0.6381806135,
0.051555071,
-0.4871641695,
0.0292484872,
-0.4754453301,
-0.4789412916,
0.2521520257,
-0.1042923704,
-0.0297281742,
-0.3696422875,
0.1922225356,
-0.4077890217,
0.3286344409,
0.102177918,
0.1139923856,
0.1136902794,
-0.1395548433,
0.0326823257,
0.3232571781,
0.2458925843,
0.0373669043,
0.047951024,
-0.0644854531,
0.0700882822,
-0.2977600098,
0.4409293234,
-0.2956631482,
0.052874919,
0.2401667237,
-0.013478484,
0.298935324,
-0.230269894,
0.1710726172,
0.3026788533,
0.5181800127,
-0.0112024993,
0.113107115,
-0.4032348394,
-0.2267827392,
-0.0801947415,
0.1673658937,
0.1750005335,
0.0717569441,
0.1927924603,
-0.3779023886,
0.125996545,
0.1591121107,
-0.045112431,
0.2188045681,
0.3621394932,
-0.3730173707,
0.2935865521,
0.1711705327,
-0.0791651681,
0.1143633425,
-0.2527514398,
-0.3305525184,
0.1376244724,
-0.2099725008,
0.0138350418,
-0.1173722893,
0.3929837346,
-0.1036164239,
0.0236905776,
0.1074152961,
-0.0235767439,
0.1798297614,
0.1378367692,
0.4080688953,
0.0065899771,
0.446965158,
0.0536669232,
-0.1491610706,
-0.1865977943,
0.1863408387,
-0.0538992286,
0.0364208817,
-0.1401544809,
0.5328085423,
0.1328556687,
0.1173180714,
-0.1514059454,
-0.0866408572,
0.0324665569,
-0.1064754501,
-0.2918033302,
0.0128358649,
0.1817287356,
-0.046955809,
-0.0455383062,
-0.1222739369,
-0.0642847344,
0.0570405535,
-0.1738078892,
-0.0831567496,
-0.3341276944,
-0.3643176258,
0.1104173958,
-0.0247189067,
-0.2077739984,
0.3294540346,
0.1876463592,
-0.1365974098,
0.0210158806,
0.1092822179,
-0.1964777112,
0.1147877276,
0.6276438832,
-0.2282990217,
0.2196656615,
0.3440071642,
0.1959387958,
-0.1997303069,
0.4353932142,
-0.4109543562,
-0.4699917138,
0.0184687618,
0.088431932,
-0.1389921606,
0.0448096469,
-0.3083497584,
0.1366657466,
0.3585319519,
0.125195086,
0.2424765378,
-0.1067553163,
-0.3246654868,
-0.1552181989,
-0.1979093403,
0.5811359286,
-0.1848370582,
-0.302549094,
-0.3280836046,
-0.4322116077,
0.2125725597,
0.1218338832,
-0.213690117,
0.280059278,
-0.3107711673,
-0.0972534716,
0.308210969,
-0.1050790325,
0.0822916776,
0.4469819367,
-0.2502986193,
0.1680026501,
0.2125219405,
0.0836040229,
-0.215675503,
-0.1224220246,
0.0135817155,
-0.4536336064,
0.0406976305,
-0.154610917,
-0.1473659426,
-0.1895033717,
0.296046257,
0.0144894347,
-0.2710359693,
-0.0140961418,
0.0367449932,
-0.1554628611,
0.239770487,
0.12712726,
0.1040885597,
0.043124117,
-0.1647825092,
0.0198250208,
-0.0450831912,
0.3280402124,
-0.6091820002,
0.0961713716,
0.1165379137,
-0.0305938981,
0.0045086201,
-0.0909323767,
-0.0490077734,
-0.0273274761,
-0.3792023957,
-0.1640515924,
-0.0221962705,
0.0016260839,
-0.05961987,
-0.0850862563,
-0.0751339644,
0.2245912254,
-0.5344882607,
0.0863711685,
0.0056908303,
-0.1661025733,
0.0117708454,
0.3507804871,
-0.2235099375,
-0.1054346338,
0.1355481595,
0.0792682543,
-0.1547690332,
0.2173432708,
0.1086671576,
0.2987657487,
-0.0428355038,
0.3947450519,
0.0307755638,
-0.199535653,
0.4566540718,
-0.1920306981,
-0.1919047683,
-0.1184583157,
0.1470096707,
0.1488820314,
-0.0293641146,
0.2458750308,
-0.3589743376,
0.0014750742,
0.0724730715,
0.1763550341,
-0.1827460527,
-0.5318388939,
-0.0535604879,
0.1382283866,
0.2236329317,
0.3524546623,
0.0328615122,
0.0614067465,
0.5036966801,
-0.0225225147,
-0.0350023583,
0.1725946218,
-0.1172598228,
-0.126762107,
0.2107432485,
0.0995992348,
0.4006418586,
0.1433573663,
0.0964036137,
0.2135553956,
0.0398834646,
-0.0287604388,
0.284573257,
0.0011581631,
0.291946888,
0.0412639864,
-0.4688801765,
-0.0534956902,
-0.2464309186,
0.0426367074,
-0.1107653677,
-0.1338510662,
-0.548420012,
-0.1352429241,
-0.1794123203,
-0.2473459989,
-0.3247793019,
-0.2180156559,
-0.2057372034,
-0.4174846709,
0.0159907974,
-0.1967617273,
-0.3559074998,
0.1864646822,
-0.1796561033,
0.2185810059,
0.1087455302,
0.270239085,
-0.3594065011,
-0.2073976547,
-0.1868629009,
0.0056630489,
-0.175374344,
-0.1987686306,
0.0720700473,
-0.3009379208,
0.2761230767,
-0.2969669104,
-0.1735820025,
0.162887156,
-0.3161105216,
-0.0158545747,
0.1541596055,
0.0007796328,
0.0341844857,
-0.1384244263,
0.0944355875,
0.1207675487,
-0.0642789826,
-0.3195793331,
0.160495311,
0.1289813817,
-0.1551725417,
-0.2121982872,
-0.3349226117,
-0.151320219,
-0.1797147989,
-0.3641606867,
0.1897164881,
0.3864743114,
0.0024571877,
0.1253542155,
-0.2772358358,
0.2045514435,
-0.3516031504,
-0.6407562494,
0.0419360362,
-0.0941986144,
0.0447055027,
0.0233053118,
0.1199382469,
0.1616581976,
-0.0717077479,
-0.8328555226,
0.0837568045,
-0.1131229624,
-0.2497928739,
0.2192756981,
-0.0311910324,
-0.0892971829,
0.2955487669,
-0.0040108529,
-0.0155309532,
-0.2937014401,
0.0342544056,
-0.4827652276,
0.2337221354,
0.0650284514,
0.4955636263,
-0.1274765879,
0.6671247482,
0.1786766201,
-0.027556058,
0.1970890015,
0.0470816381,
0.6038402915,
-0.1499834061,
-0.1548582017,
-0.111225076,
0.0743589625,
-0.1259298027,
-0.0157107301,
-0.084140785,
0.0476633348,
0.1504990757,
0.1654736847,
0.0786561146,
-0.24259606,
0.0733594671,
-0.4269170463,
-0.0912576318,
-0.144654274,
0.073991105,
0.1281628311,
-0.0123729017,
0.011063382,
0.4801498652,
0.3755213022,
0.0773746669,
-0.2766480744,
-0.1589406282,
-0.1690035015,
0.1122554541,
0.0917743742,
0.0670728013,
-0.3882783651,
-0.306481868,
-0.0721234307,
0.3549326956,
0.1371837854,
-0.1570130289,
0.2949313521,
0.13180466,
-0.1216842979,
-0.1347220689,
-0.2034214586,
0.0879287496,
0.1541792303,
0.1276381612,
0.171755597,
-0.083869718,
-0.396053642,
0.1100464389,
-0.1419894993,
-0.1392143369,
-0.1915452331,
-0.2848085165,
0.2992539108,
-0.2038094401,
-0.003204691,
-0.0412968174,
-0.1334178299,
-0.4892788529,
-0.1212308034,
0.1779164821,
0.2980812192,
-0.0719582289,
-0.1004739553,
-0.0188297946,
0.3366836011,
-0.0939927921,
0.3638508618,
0.6472287178,
0.2555454969,
0.7740369439,
0.0363735817,
-0.1040385514,
-0.0139202382,
-0.0484958515,
0.2307561636,
0.1523725986,
-0.0274608601,
0.0000713354,
0.3212533593,
0.3440135717,
-0.4421432018,
0.1337493956,
0.231380254,
0.1668225676,
-0.4083358645,
-0.2868037224,
0.5116582513,
0.2142746001,
-0.104391858,
0.5442041159,
0.0711136833,
-0.1615946889,
-0.2187599838,
0.3741799295,
0.8942178488,
-0.1471622437,
0.1002799273,
-0.0160289556,
-0.0071232864,
0.1717580855,
0.1440544426,
-0.2678990662,
0.007318439,
0.1029143929,
-0.09251073,
-0.0707772523,
0.1881533861,
0.52908355,
-0.1787946522,
0.0160601083,
-0.2433733791,
0.3730197251,
0.0617843457,
-0.0121213598,
-0.0502513535,
-0.093608059,
-0.1660183519,
0.0052701645,
0.1045098901,
0.2041030824,
-0.2394822538,
0.0460052378,
0.379873842,
-0.2689360976,
-0.1060882062,
-0.2011126727,
-0.2168131173,
0.1850458533,
-0.0572736487,
-0.0470173061,
0.1191345751,
0.0937592164,
-0.0234392099,
0.1446026266,
0.0119471774,
-0.1187983155,
0.0615762994,
0.0238211676,
-0.3643184006,
0.0179008432,
0.2325510681,
-0.0072844927,
-0.2646377385,
0.204837516,
-0.3840930164,
-0.5043624043,
0.0228942055,
0.0711932182,
-0.1922389269,
-0.203635633,
0.0533128865,
0.1699160784,
-0.2309155315,
0.2873207629,
0.0293757785,
0.1502048522,
0.1965529621,
0.0601567551,
0.2314126194,
-0.648314774,
-0.1805449724,
0.2899326682,
0.0787511691,
-0.0787154883,
0.4794631898,
-0.2117161006,
-0.0789495111,
-0.139301464,
0.0622333623,
0.3695783317,
-0.0275950637,
-0.1137567759,
0.3495696783,
0.0395735055,
0.1129498184,
-0.025881514,
0.2895483673,
0.2128801346,
-0.2625929713,
-0.3203586638,
-0.6266812682,
0.2629425824,
-0.5137577653,
0.1910709441,
-0.14088054,
-0.2451644093,
0.303681314,
0.1079545915,
-0.1916730404,
-0.0999564454,
0.0556895547,
-0.0071933307,
0.1709096134,
0.1493301243,
0.3320842087,
0.2791548371,
-0.0922304168,
-0.3216713369,
-0.4433017075,
-0.040005561,
0.1684955508,
0.2169547528,
0.0094912397,
-0.0795173794,
-0.2575776279,
-0.1280805767,
0.101952821,
-0.0778398365,
0.3905067444,
0.1049129963,
-0.0095972288,
0.3817889094,
-0.1426332146,
-0.0086786691,
-0.2456884682,
-0.0357541814,
0.1109166592,
0.0550187342,
-0.1513473839,
-0.1801465899,
-0.0491044894,
-0.0539738536,
0.2511946261,
0.0999223962,
0.0972090065,
-0.078599751,
0.1991379112,
0.1278098226,
0.1732059866,
0.2066396326,
0.1552706808,
0.055915568,
0.0019711482,
-0.3858050704,
-0.2215706408,
0.0646760464,
0.0356071964,
0.0378213786,
-0.1296640038,
0.4016624987,
0.00598269,
0.0710970163,
0.1350275874,
0.1085011661,
0.550332129,
0.1401060224,
0.1703459322,
-0.4319105744,
0.2125663906,
0.3312864602,
-0.2809247971,
0.3473609984,
0.5354616046,
0.1322885603,
0.2809292674,
0.0803070664,
0.2377754003,
0.5244954824,
0.3298661411,
0.0640480965,
-0.1033365428,
-0.5520820618,
0.101558134,
0.6678977609,
-0.5076913238,
-0.1573925465,
0.1145320982,
0.0160785429,
0.1352502257,
-0.1314965636,
-0.1689446419,
-0.1418943703,
-0.1557699442,
-0.0667337179,
0.094025746,
-0.0192205645,
-0.0575184077,
-0.0362388492,
0.1004228666,
-0.0434548967,
0.2444916964,
-0.3040907681,
-0.254470706,
-0.0272781476,
-0.0317718424,
0.2784423232,
0.1199141592,
-0.2305404395,
-0.0807029828,
0.1579209566,
-0.0214182474,
0.4265594184,
0.5706606507,
0.5717046857,
0.6063529849,
0.1830432713,
0.1278857589,
0.0131118745,
0.0590676777,
-0.0073183742,
0.6166631579,
-0.0045634471,
-0.5439006686,
0.2784528434,
0.0439493805,
0.0041392967,
0.3585560322,
-0.2119916826,
0.3224887848,
0.1105725989,
0.1054096073,
0.064269878,
-0.0584098995,
0.1374061704,
0.0827972516,
-0.2589647174,
0.0726366341,
0.9143494368,
-0.1347180456,
-0.0051504239,
-0.3627816737,
0.0297407694,
0.0700254589,
0.7529671788,
0.6106792688,
0.0658987388,
-0.3105234802,
0.0288969874,
-0.5796037316,
0.082353361,
-0.0141897425,
0.3293287456,
0.198584497,
-0.215730533,
0.1154161096,
0.3421983719,
0.2262533903,
-0.3068059683,
-0.0698385835,
-0.4682078958,
-0.2701193988,
-0.0169811584,
0.345426023,
0.1913651228,
-0.066605024,
-0.1096358299,
0.3333044648,
-0.0088536115,
-0.058482077,
-0.1018457636,
0.2546592951,
0.0168322045,
0.4844088256,
0.363651365,
0.1958842129,
0.3726077676,
0.143644765,
0.0181053225,
-0.345630765,
-0.2510229349,
0.0901650265,
0.2232735753,
0.1879864484,
0.1458601803,
-0.154279381,
-0.038201876,
-0.2758580446,
0.0652721822,
0.0706901029,
0.0428654775,
0.1385470629,
-0.0437366441,
-0.1341781169,
-0.1813511252,
-0.0378306955,
0.1209152788,
0.495134145,
0.1649115235,
-0.0142049603,
-0.2301948369,
0.3458403945,
-0.177703023,
0.0022800583,
-0.0202444028,
0.1730800569,
0.1003088132,
-0.2233656049,
-0.8071295023,
0.0816918537,
0.4822100997,
-0.2035748363,
-0.1766885966,
0.0255612656,
-0.0607890524,
-0.0086672772,
0.0344970152,
0.4376495779,
-0.1060258076,
-0.0757306814,
-0.2217498869,
-0.5205209851
]
|
https://github.com/huggingface/datasets/issues/1948 | dataset loading logger level | Thank you for explaining the intention, @lhoestq
1. Could it be then made more human-friendly? Currently the hex gibberish tells me nothing of what's really going on. e.g. the following is instructive, IMHO:
```
WARNING: wmt16/ro-en/train dataset was loaded from cache instead of being recomputed
WARNING: wmt16/ro-en/validation dataset was loaded from cache instead of being recomputed
WARNING: wmt16/ro-en/test dataset was loaded from cache instead of being recomputed
```
note that it removes the not so useful hex info and tells the user instead which split it's referring to - but probably no harm in keeping the path if it helps the debug. But the key is that now the warning is telling me what it is it's warning me about.
```
Warning:Loading cache path
```
on the other hand isn't telling what it is warning about.
And I still suggest this is INFO level, otherwise you need to turn all 'using cache' statements to WARNING to be consistent. The user is most likely well aware the cache is used for models, etc. So this feels very similar.
2. Should there be a way for a user to void warranty by having a flag - `I know I'm expecting the cached version to load if it's available - please do not warn me about it=True`
To explain the need: Warnings are a problem, they constantly take attention away because they could be the harbinger of a problem. Therefore I prefer not to have any warnings in the log, and if I get any I usually try to deal with those so that my log is clean.
It's less of an issue for somebody doing long runs. It's a huge issue for someone who does a new run every few minutes and on the lookout for any potential problems which is what I have been doing a lot of integrating DeepSpeed and other things. And since there are already problems to deal with during the integration it's nice to have a clean log to start with.
I hope my need is not unreasonable and I was able to explain it adequately.
Thank you. | on master I get this with `--dataset_name wmt16 --dataset_config ro-en`:
```
WARNING:datasets.arrow_dataset:Loading cached processed dataset at /home/stas/.cache/huggingface/datasets/wmt16/ro-en/1.0.0/9dc00622c30446e99c4c63d12a484ea4fb653f2f37c867d6edcec839d7eae50f/cache-2e01bead8cf42e26.arrow
WARNING:datasets.arrow_dataset:Loading cached processed dataset at /home/stas/.cache/huggingface/datasets/wmt16/ro-en/1.0.0/9dc00622c30446e99c4c63d12a484ea4fb653f2f37c867d6edcec839d7eae50f/cache-ac3bebaf4f91f776.arrow
WARNING:datasets.arrow_dataset:Loading cached processed dataset at /home/stas/.cache/huggingface/datasets/wmt16/ro-en/1.0.0/9dc00622c30446e99c4c63d12a484ea4fb653f2f37c867d6edcec839d7eae50f/cache-810c3e61259d73a9.arrow
```
why are those WARNINGs? Should be INFO, no?
warnings should only be used when a user needs to pay attention to something, this is just informative - I'd even say it should be DEBUG, but definitely not WARNING.
Thank you.
| 351 | dataset loading logger level
on master I get this with `--dataset_name wmt16 --dataset_config ro-en`:
```
WARNING:datasets.arrow_dataset:Loading cached processed dataset at /home/stas/.cache/huggingface/datasets/wmt16/ro-en/1.0.0/9dc00622c30446e99c4c63d12a484ea4fb653f2f37c867d6edcec839d7eae50f/cache-2e01bead8cf42e26.arrow
WARNING:datasets.arrow_dataset:Loading cached processed dataset at /home/stas/.cache/huggingface/datasets/wmt16/ro-en/1.0.0/9dc00622c30446e99c4c63d12a484ea4fb653f2f37c867d6edcec839d7eae50f/cache-ac3bebaf4f91f776.arrow
WARNING:datasets.arrow_dataset:Loading cached processed dataset at /home/stas/.cache/huggingface/datasets/wmt16/ro-en/1.0.0/9dc00622c30446e99c4c63d12a484ea4fb653f2f37c867d6edcec839d7eae50f/cache-810c3e61259d73a9.arrow
```
why are those WARNINGs? Should be INFO, no?
warnings should only be used when a user needs to pay attention to something, this is just informative - I'd even say it should be DEBUG, but definitely not WARNING.
Thank you.
Thank you for explaining the intention, @lhoestq
1. Could it be then made more human-friendly? Currently the hex gibberish tells me nothing of what's really going on. e.g. the following is instructive, IMHO:
```
WARNING: wmt16/ro-en/train dataset was loaded from cache instead of being recomputed
WARNING: wmt16/ro-en/validation dataset was loaded from cache instead of being recomputed
WARNING: wmt16/ro-en/test dataset was loaded from cache instead of being recomputed
```
note that it removes the not so useful hex info and tells the user instead which split it's referring to - but probably no harm in keeping the path if it helps the debug. But the key is that now the warning is telling me what it is it's warning me about.
```
Warning:Loading cache path
```
on the other hand isn't telling what it is warning about.
And I still suggest this is INFO level, otherwise you need to turn all 'using cache' statements to WARNING to be consistent. The user is most likely well aware the cache is used for models, etc. So this feels very similar.
2. Should there be a way for a user to void warranty by having a flag - `I know I'm expecting the cached version to load if it's available - please do not warn me about it=True`
To explain the need: Warnings are a problem, they constantly take attention away because they could be the harbinger of a problem. Therefore I prefer not to have any warnings in the log, and if I get any I usually try to deal with those so that my log is clean.
It's less of an issue for somebody doing long runs. It's a huge issue for someone who does a new run every few minutes and on the lookout for any potential problems which is what I have been doing a lot of integrating DeepSpeed and other things. And since there are already problems to deal with during the integration it's nice to have a clean log to start with.
I hope my need is not unreasonable and I was able to explain it adequately.
Thank you. | [
-0.1465869844,
-0.2024427652,
0.0129190655,
0.3102097511,
0.4003247619,
0.3999479413,
0.4905202687,
0.2311709076,
0.1243601888,
0.0282331128,
0.0093347831,
-0.1472629607,
-0.2483209074,
-0.1882983148,
-0.2508572936,
0.0609390996,
-0.1264946014,
-0.0315524377,
-0.3859975934,
-0.1280376911,
0.0110196648,
-0.0093770614,
0.0819808766,
0.4615404904,
-0.6953532696,
-0.05773101,
0.0907773823,
0.2692827582,
0.0484168865,
-0.704439342,
0.268795073,
-0.061060816,
0.1792587191,
0.1538810879,
-0.0001220934,
0.1301618218,
0.6268300414,
0.0067863967,
-0.6243700981,
0.0161632933,
-0.3979128003,
-0.3823041916,
0.1909535974,
-0.0893725902,
-0.0878833681,
-0.3329227269,
0.3174684346,
-0.3982445002,
0.3191030324,
0.1539320499,
0.0972731858,
0.1309638768,
-0.1569448113,
0.1304769218,
0.3219231069,
0.2671613097,
-0.0668996796,
0.0625436157,
-0.0271569267,
0.1869477481,
-0.3544818759,
0.3654178977,
-0.1897871792,
-0.0355945192,
0.1682561338,
-0.0251547024,
0.2707445323,
-0.0829855651,
0.0980233699,
0.457020998,
0.4693350494,
-0.1381200701,
0.0960090309,
-0.3951649368,
-0.1142803803,
-0.3058126271,
0.1722684503,
0.1817940772,
-0.1236341745,
0.1642874777,
-0.278645575,
0.096231021,
0.0633186325,
-0.0412001647,
0.2753939033,
0.5356915593,
-0.2549029589,
0.199491024,
0.2321577221,
-0.1086487994,
0.3180998266,
-0.0647456571,
-0.2923905849,
0.055742383,
-0.3284549117,
0.0961240754,
-0.1569817066,
0.2279084921,
-0.1270325631,
0.0550278351,
0.0303927828,
0.0645742938,
0.1766625643,
0.0465370491,
0.3021318316,
0.0782382786,
0.3390366137,
-0.0437264442,
-0.2039044201,
-0.0232510976,
0.1906761825,
-0.052289106,
-0.1306730807,
-0.0884444639,
0.4426143765,
0.2055937648,
0.0061231614,
-0.0329379737,
-0.1966417283,
0.0259853769,
-0.1524892598,
-0.3309822083,
0.0248685759,
0.1910765171,
-0.0790169761,
-0.0314244777,
-0.1334367245,
-0.0265854094,
-0.0438803174,
-0.2736994624,
-0.0795498192,
-0.2813802361,
-0.3722064793,
0.17711474,
-0.1128014475,
-0.1633093655,
0.1749127954,
0.178488642,
-0.0563447811,
0.0769141093,
-0.0438766815,
-0.1474411339,
0.1251859665,
0.4888394773,
-0.1188972518,
0.2109149843,
0.3153441548,
0.1144106686,
-0.2508602142,
0.3219142556,
-0.3088694811,
-0.4429716766,
-0.1388502121,
0.0568971895,
-0.1872851849,
-0.0427055843,
-0.2263442874,
0.2049742937,
0.2080991864,
0.2616477609,
0.3043172359,
-0.0870517269,
-0.2893234491,
-0.1400416791,
-0.1068018749,
0.604522109,
-0.2300406694,
-0.3448927402,
-0.2233901769,
-0.3908490837,
0.3220081329,
0.1940955967,
-0.1252970397,
0.3429294229,
-0.2669638693,
-0.1275547743,
0.2065178305,
-0.1993076354,
0.1538256705,
0.5722748041,
-0.2666298449,
0.3573939502,
0.2487149984,
0.0757365152,
-0.3795432746,
-0.1587415934,
0.0931628495,
-0.4061235487,
0.0346498452,
-0.2195474952,
-0.1422886848,
-0.3101748228,
0.3162403703,
0.0956734642,
-0.1243339181,
-0.040762607,
-0.0270058159,
-0.059659034,
0.5715070963,
0.0659454018,
0.0881292224,
0.0159738977,
-0.0474754162,
0.0137910703,
-0.1247670799,
0.3047451973,
-0.4733554423,
0.0033311895,
0.2965785563,
0.1541423649,
0.0607187115,
-0.0349124707,
-0.1101766452,
-0.0416643992,
-0.4651913047,
-0.1592129618,
-0.0148240002,
0.0448276848,
-0.2315160483,
-0.1339941323,
-0.122830905,
0.3283224106,
-0.5877946019,
0.0591800921,
-0.1173663512,
-0.3510157168,
0.0371699706,
0.3243011534,
-0.2366800457,
0.0116763096,
0.1059906036,
0.0001265435,
-0.1354236156,
0.2674869895,
-0.0427936465,
0.3116447031,
-0.0823950022,
0.541757822,
0.0388026722,
-0.1560538262,
0.3681596518,
-0.2089683264,
-0.2602341771,
-0.0510698035,
0.1137241051,
0.026450051,
0.0294060707,
0.2619309425,
-0.2610863745,
-0.106624864,
0.0779919773,
0.0519059598,
-0.1736051589,
-0.3629190624,
0.1012514234,
0.1045231372,
0.2224513143,
0.3565241396,
-0.0605903268,
0.1401377022,
0.3738935888,
-0.0226564668,
-0.000953759,
0.2139961272,
-0.0660069287,
-0.1223925278,
0.1966654211,
0.0896257088,
0.1997383535,
0.1502247602,
0.1785116941,
0.2778915763,
-0.0631351396,
-0.0572557785,
0.257706821,
0.0119199036,
0.4047443569,
0.0357545763,
-0.4561923146,
0.0109884897,
-0.2117696255,
-0.0841504186,
-0.1447160095,
-0.0529185012,
-0.6462906003,
0.0031947491,
-0.2683838308,
-0.343390733,
-0.3749731779,
-0.2526738644,
-0.1563919187,
-0.3785589337,
0.008687987,
-0.3024222255,
-0.3954235911,
0.1360272318,
-0.3061368465,
0.2309262007,
0.100167267,
0.2513025403,
-0.2852824926,
-0.0661874339,
-0.2680497169,
-0.0152586959,
-0.0835172161,
-0.2519968748,
0.0985289887,
-0.4905314445,
0.1629212499,
-0.1752011329,
-0.202642113,
0.308850795,
-0.1975003928,
0.0879949108,
0.1897614747,
-0.0893783793,
-0.0432893559,
-0.2671487033,
0.0250471607,
0.1185784191,
0.0083138011,
-0.3542829156,
0.0778484568,
0.1457301825,
-0.1193104982,
-0.0913557932,
-0.3239559531,
-0.1959174722,
-0.127250731,
-0.3672882318,
0.2959489524,
0.4755592942,
0.0311138034,
0.0013715253,
-0.1817463487,
0.2356294543,
-0.2804740369,
-0.7693063617,
-0.0325261243,
-0.1196925268,
0.1096624285,
-0.0435091518,
0.1002776846,
0.1628789157,
0.0031549872,
-0.8037421703,
0.1855157465,
-0.1419695914,
-0.3532153368,
0.1603693962,
-0.0036665692,
-0.1876033396,
0.2181838453,
-0.0146572851,
0.0057899472,
-0.286221087,
0.0531044416,
-0.46766451,
0.4226560295,
0.1411722004,
0.4266451001,
0.1103249937,
0.4797786772,
0.1754453331,
-0.0096642906,
0.2206351608,
-0.04050624,
0.6518880725,
-0.0414569862,
-0.0822482407,
0.0396548137,
0.0212177783,
-0.2239886969,
-0.0989488661,
-0.0745001063,
0.2100463063,
0.2413642257,
0.0937355384,
0.0352377743,
-0.4016299248,
0.2715889513,
-0.4244951606,
-0.1394437999,
-0.1997303367,
0.1225216538,
0.2278077602,
-0.0328869633,
0.1564408988,
0.4967170954,
0.2978890538,
0.1715158373,
-0.2740751207,
-0.1311542094,
-0.3372916579,
-0.0180855468,
0.0550626293,
0.161672473,
-0.3739034235,
-0.3055793047,
-0.110557735,
0.3051780462,
0.1360484958,
-0.1391133964,
0.2344478071,
0.1739259511,
-0.0696469396,
-0.0882602707,
-0.1640913486,
0.0512594059,
0.0295519587,
0.1467776597,
0.0859368816,
-0.1525841653,
-0.4390390217,
0.1397967637,
-0.096128054,
-0.2378574163,
-0.250474304,
-0.2054908276,
0.1677543819,
-0.0954987854,
-0.0245509949,
-0.0470244773,
-0.0282837562,
-0.5195002556,
-0.0082281632,
0.1157223731,
0.3064213395,
0.0755930543,
-0.1433746815,
-0.0902580693,
0.3614207804,
-0.1069242656,
0.432739377,
0.704102397,
0.2550819516,
0.729955256,
0.0712156519,
-0.0811749622,
-0.0565038845,
-0.0520723462,
0.2179765254,
0.3498032093,
0.1193917319,
-0.0570037216,
0.3241609037,
0.4321882129,
-0.4970782697,
0.3054848313,
0.2432099283,
0.1004248485,
-0.4775583446,
-0.3319885731,
0.4986375272,
0.1059213951,
-0.0401843414,
0.4706760347,
0.2508617938,
-0.1733492166,
-0.1791163385,
0.448597759,
1.0204640627,
-0.1413293332,
0.2379665226,
0.0887255073,
-0.0671918914,
0.2032372504,
0.0498396754,
-0.2287022471,
-0.0339879878,
0.0954980627,
-0.1102619693,
-0.0079032863,
0.0217751302,
0.4259311259,
-0.0814968944,
0.0552997217,
-0.3799415231,
0.199765265,
0.2381652892,
0.0111749582,
-0.1948654056,
-0.1852942109,
-0.1854829192,
-0.0109014856,
0.19295533,
0.1259742677,
-0.254964292,
-0.0789037496,
0.3553370237,
-0.1292887926,
-0.205978334,
-0.1289225668,
-0.2180996388,
-0.0243699085,
-0.1056507006,
-0.0971039012,
0.024286529,
-0.0689305216,
0.0443327986,
0.1664552838,
0.0667943284,
0.0058087455,
0.0035963894,
0.0768847615,
-0.316405654,
-0.0807072148,
0.3426614404,
0.0499624498,
-0.3006898165,
0.2033225745,
-0.2766221464,
-0.5778277516,
0.051915355,
-0.1594388932,
-0.2073709667,
-0.0885657668,
0.139916122,
0.1879904717,
-0.1414647698,
0.221740976,
0.0167124569,
0.1536529213,
0.2042516768,
0.0143992258,
0.2500065863,
-0.5792985559,
-0.1628117561,
0.0786971748,
0.0129219955,
-0.1310415417,
0.4648007452,
-0.0984343663,
-0.0492203161,
-0.1144693494,
-0.0070029916,
0.5963893533,
-0.1043780074,
-0.1934036463,
0.2725799382,
-0.0487125628,
0.0769601986,
-0.1061723828,
0.3125025034,
0.0999435484,
-0.2827931643,
-0.226707831,
-0.5409590006,
0.2808479071,
-0.4361149967,
0.212540701,
-0.1690122187,
-0.1476345509,
0.2259997129,
0.0833065435,
-0.1783975363,
-0.2111174911,
-0.0559465066,
-0.1625298858,
0.1657116413,
0.2391403168,
0.3696459532,
0.2524447441,
-0.0903136581,
-0.3158457577,
-0.3205566704,
-0.0216380917,
-0.0654083863,
0.2244133949,
-0.0104354015,
-0.1175985411,
-0.0966224149,
-0.0900587738,
0.1384565234,
-0.182805866,
0.3072933853,
0.2030452043,
0.0739669874,
0.4574137926,
-0.2511948943,
0.1956443042,
-0.3103451729,
-0.0566113889,
0.3130632639,
0.2139308602,
-0.105152905,
-0.1277211756,
-0.0128360623,
-0.0179276895,
0.3755548596,
0.1243967265,
0.2455983013,
-0.0501768142,
0.0954121798,
0.2405619025,
0.1526502073,
0.173677668,
0.2529512346,
0.1828891039,
-0.0151579622,
-0.4614329636,
-0.2064891011,
0.0549772531,
0.0874795541,
0.0284562726,
-0.3894965649,
0.3267394602,
-0.0016324384,
0.1633828878,
0.1570383906,
0.1106399596,
0.4825290143,
-0.0165399238,
0.0794720501,
-0.4653485715,
0.1203660071,
0.3244591653,
-0.3549874127,
0.275141716,
0.4656181335,
-0.0184546206,
0.3258893788,
0.2565748394,
0.3138429523,
0.5164313316,
0.3635669947,
0.1383248568,
-0.1878061742,
-0.4322496951,
0.0178329982,
0.5528191924,
-0.3737701178,
-0.0973557979,
0.1278448403,
0.0378068648,
0.2741697133,
-0.1087609157,
-0.1625674963,
-0.2601648271,
-0.0821955651,
-0.1250254363,
0.054807242,
-0.0606840849,
-0.2160612494,
-0.0553319678,
0.0889470503,
-0.1751571298,
0.1543831676,
-0.2759081125,
-0.1503822356,
-0.082684271,
-0.0239451136,
0.3113577664,
0.1572371125,
-0.2301955968,
-0.172121644,
0.3310584128,
-0.1155634895,
0.4097521603,
0.4752764404,
0.6177264452,
0.5910734534,
0.1977445632,
0.141023308,
-0.0959320068,
0.0992012322,
-0.0008838087,
0.4664738476,
0.0532715805,
-0.5392205715,
0.3291277289,
0.0190835204,
-0.0180720221,
0.4304613471,
-0.1307461113,
0.3070870638,
0.0956979617,
0.1636206061,
0.0522705056,
0.0454725996,
0.0786596611,
0.1035525948,
-0.2113203853,
0.0828135982,
0.9799098969,
-0.2187812924,
0.0082386704,
-0.3598149717,
0.0376449898,
0.1337390542,
0.7504999638,
0.530143261,
0.0191349089,
-0.2689057887,
0.1098714098,
-0.678555727,
0.161548987,
-0.0100107025,
0.1195641831,
0.2431478798,
-0.1740595847,
0.1362669021,
0.2347295731,
0.2257976234,
-0.3438851237,
0.0795261189,
-0.3624972999,
-0.2689085305,
0.0313594081,
0.4597536027,
0.2654233575,
-0.0453867018,
-0.1311107427,
0.2973899841,
0.0306784995,
-0.0711345151,
-0.1218312681,
0.1676904112,
0.1573792547,
0.3829619586,
0.3445104361,
0.1535736024,
0.3686423004,
0.0324401371,
-0.1236779466,
-0.4942080379,
-0.1948025674,
-0.0650608167,
0.2983180881,
0.1826140136,
-0.0177901108,
-0.0968003497,
0.0101348739,
-0.3805669248,
0.1060071737,
0.061048843,
0.0909547582,
-0.0067865164,
0.1168816537,
-0.1358055919,
-0.0998945981,
0.0673668534,
0.1554570347,
0.5111299753,
0.1131734848,
0.0115883695,
-0.2730743289,
0.2586713433,
-0.0700675175,
-0.050063245,
-0.1905663013,
0.1469298154,
0.2262372971,
-0.0769286603,
-0.8204526305,
0.1293431967,
0.3578500748,
-0.156428203,
-0.1171126217,
0.1346118152,
-0.0860334933,
0.006534135,
0.0855475068,
0.4466447532,
-0.0737442151,
-0.1286572665,
-0.1838978976,
-0.5174062848
]
|
https://github.com/huggingface/datasets/issues/1948 | dataset loading logger level | Hey, any news about the issue? So many warnings when I'm really ok with the dataset not being recomputed :) | on master I get this with `--dataset_name wmt16 --dataset_config ro-en`:
```
WARNING:datasets.arrow_dataset:Loading cached processed dataset at /home/stas/.cache/huggingface/datasets/wmt16/ro-en/1.0.0/9dc00622c30446e99c4c63d12a484ea4fb653f2f37c867d6edcec839d7eae50f/cache-2e01bead8cf42e26.arrow
WARNING:datasets.arrow_dataset:Loading cached processed dataset at /home/stas/.cache/huggingface/datasets/wmt16/ro-en/1.0.0/9dc00622c30446e99c4c63d12a484ea4fb653f2f37c867d6edcec839d7eae50f/cache-ac3bebaf4f91f776.arrow
WARNING:datasets.arrow_dataset:Loading cached processed dataset at /home/stas/.cache/huggingface/datasets/wmt16/ro-en/1.0.0/9dc00622c30446e99c4c63d12a484ea4fb653f2f37c867d6edcec839d7eae50f/cache-810c3e61259d73a9.arrow
```
why are those WARNINGs? Should be INFO, no?
warnings should only be used when a user needs to pay attention to something, this is just informative - I'd even say it should be DEBUG, but definitely not WARNING.
Thank you.
| 20 | dataset loading logger level
on master I get this with `--dataset_name wmt16 --dataset_config ro-en`:
```
WARNING:datasets.arrow_dataset:Loading cached processed dataset at /home/stas/.cache/huggingface/datasets/wmt16/ro-en/1.0.0/9dc00622c30446e99c4c63d12a484ea4fb653f2f37c867d6edcec839d7eae50f/cache-2e01bead8cf42e26.arrow
WARNING:datasets.arrow_dataset:Loading cached processed dataset at /home/stas/.cache/huggingface/datasets/wmt16/ro-en/1.0.0/9dc00622c30446e99c4c63d12a484ea4fb653f2f37c867d6edcec839d7eae50f/cache-ac3bebaf4f91f776.arrow
WARNING:datasets.arrow_dataset:Loading cached processed dataset at /home/stas/.cache/huggingface/datasets/wmt16/ro-en/1.0.0/9dc00622c30446e99c4c63d12a484ea4fb653f2f37c867d6edcec839d7eae50f/cache-810c3e61259d73a9.arrow
```
why are those WARNINGs? Should be INFO, no?
warnings should only be used when a user needs to pay attention to something, this is just informative - I'd even say it should be DEBUG, but definitely not WARNING.
Thank you.
Hey, any news about the issue? So many warnings when I'm really ok with the dataset not being recomputed :) | [
-0.2018210739,
-0.2147749513,
-0.0191504974,
0.4123525918,
0.4625154436,
0.4148596227,
0.4687034786,
0.127430439,
0.1240054592,
-0.0095587233,
0.0188383516,
-0.0816432834,
-0.2626186013,
-0.1146503836,
-0.3601995111,
0.256483376,
-0.0692486316,
-0.0352245457,
-0.4280804396,
-0.0789847001,
-0.1100228205,
0.0888151601,
-0.0381994359,
0.4029662907,
-0.7883704305,
-0.0844628438,
0.114155598,
0.0337204412,
-0.014049612,
-0.7113649249,
0.271253258,
-0.0159590244,
0.1750288904,
0.1797814518,
-0.0001194563,
0.0807434022,
0.5874012709,
0.0346958824,
-0.5454555154,
0.0874001309,
-0.3945809603,
-0.4607205689,
0.2950289547,
0.0680097342,
-0.0818455219,
-0.4350314736,
0.105390422,
-0.4060836136,
0.3432333171,
0.0942690596,
0.1741232425,
0.1127503365,
-0.0637700781,
0.0258959122,
0.2801208198,
0.2445057631,
-0.0443210229,
0.0054730102,
0.0054391618,
0.0546715558,
-0.3033307493,
0.4369639754,
-0.1826980263,
0.0547143333,
0.1919062287,
-0.0574978665,
0.2136777043,
-0.1872317642,
0.1827514023,
0.3480859697,
0.5612228513,
0.0119368332,
-0.0213174783,
-0.4384615123,
-0.1327172965,
-0.2757674158,
0.162834987,
0.2771093547,
0.0638033152,
0.2064714879,
-0.3305914104,
0.0531377718,
0.1248336285,
-0.1494836658,
0.136418134,
0.2955520153,
-0.4321041703,
0.1811941117,
0.1696337312,
-0.0837929174,
0.1944062561,
-0.1643509716,
-0.3497732282,
0.0897096321,
-0.3535855412,
0.0463142246,
-0.1576974094,
0.4689551294,
-0.073959142,
0.2159976661,
0.0167460926,
0.0333990715,
0.168204084,
-0.0259478725,
0.4356086254,
0.0151562467,
0.308208406,
-0.0288094208,
-0.1062848493,
-0.1515375972,
0.1799235493,
-0.0751505569,
0.031502191,
-0.123455815,
0.4663627446,
0.0360623896,
0.1819915771,
-0.2072842568,
-0.2261763364,
0.0814375356,
-0.0244263168,
-0.2710514963,
0.0001725607,
0.1685473621,
-0.0864167288,
0.0307929907,
-0.095915772,
-0.0316217355,
-0.0068022609,
-0.2094553113,
-0.1628877968,
-0.3538590968,
-0.3196652234,
0.1527387947,
0.0468781404,
-0.2135723084,
0.2720472813,
0.2136950046,
-0.0537762567,
0.0015834415,
0.0000410583,
-0.1857968569,
0.0291505847,
0.5929058194,
-0.2160653919,
0.2531104088,
0.2836387455,
0.2028167844,
-0.1459083855,
0.3028618097,
-0.3097998202,
-0.5017564893,
-0.0863300562,
0.1424662173,
-0.213052392,
-0.0198233575,
-0.2632067204,
0.0765114948,
0.3247368336,
0.2773108184,
0.1310300082,
-0.1277331263,
-0.2539334893,
-0.1192452013,
-0.1299890578,
0.5831536055,
-0.1704382747,
-0.22820279,
-0.3527734578,
-0.3691098988,
0.2504703403,
0.1459609419,
-0.1416141987,
0.2105037421,
-0.3315040767,
-0.2182202488,
0.1891389042,
-0.0765953511,
0.0075309961,
0.4234817326,
-0.1885272861,
0.1410766989,
0.2532602549,
0.0574840792,
-0.2100538164,
-0.1748189032,
-0.0686212853,
-0.3766585588,
0.0073148054,
-0.1962322444,
-0.127981171,
-0.2532562017,
0.3050476313,
0.0753608346,
-0.2619209886,
-0.0613041259,
-0.0347080082,
-0.1696526259,
0.287730068,
0.1290986985,
0.1004645899,
0.0401726216,
-0.0632457435,
0.0750576779,
-0.0070596845,
0.29626441,
-0.6046151519,
0.0570385493,
0.2527225912,
0.028345665,
-0.0281587448,
-0.0778325871,
-0.0409897417,
0.0045938389,
-0.4720385671,
-0.2500115335,
0.0077039194,
0.0479584262,
-0.1238508597,
-0.1356067508,
-0.1704798937,
0.3103653193,
-0.56722188,
0.1139789298,
0.0017966579,
-0.1301008016,
0.0311942212,
0.2780260444,
-0.0537166521,
-0.003340415,
0.0597092323,
0.09261702,
-0.1194308922,
0.171471864,
0.0795503482,
0.3461109698,
-0.0664778426,
0.348244369,
0.0421642885,
-0.22758919,
0.3439952731,
-0.1905630529,
-0.1876618266,
-0.0942143574,
0.1217284873,
0.1971225888,
-0.0955973342,
0.2936930358,
-0.2681663334,
-0.0041470174,
0.1174797043,
0.1158287078,
-0.1516928077,
-0.5100905299,
0.0351174474,
0.117378056,
0.255050391,
0.3448005021,
-0.0433747657,
0.1233632341,
0.4002453387,
0.0338242203,
-0.0325984135,
0.1823849976,
-0.105986841,
-0.0716443434,
0.1637207121,
0.1360665262,
0.3655403256,
0.199943319,
0.0699334145,
0.1784567088,
0.0452741012,
-0.0914444923,
0.3495110571,
-0.0858701617,
0.3064245284,
0.1464741379,
-0.391076237,
-0.0546412542,
-0.3296444416,
0.112201646,
-0.0881776735,
-0.0445915572,
-0.5437099338,
-0.1114683449,
-0.3159331679,
-0.2503341138,
-0.4220112562,
-0.2341748029,
-0.2240232825,
-0.391638577,
0.0054607894,
-0.1400474161,
-0.3002393246,
0.19751589,
-0.1929399967,
0.245929569,
0.0707639456,
0.3600009978,
-0.3217202723,
-0.1065908819,
-0.2888939679,
0.0322102793,
-0.0777423903,
-0.2631669641,
0.2102136016,
-0.2736949325,
0.2459833026,
-0.3104974926,
-0.1889105588,
0.1447821409,
-0.2983018458,
0.096301876,
0.1681462377,
-0.0129314801,
0.0876922682,
-0.2143980414,
0.0786361396,
0.1471221,
-0.0858601779,
-0.356882751,
0.1054936349,
0.1841019988,
-0.0835093856,
-0.2449281365,
-0.2904905379,
-0.1300165057,
-0.1924383938,
-0.3609961569,
0.1438361555,
0.3930368125,
-0.0703909472,
0.0316413678,
-0.254349947,
0.1763506532,
-0.3930454552,
-0.592974782,
0.0932761431,
-0.0530359671,
-0.0479281507,
0.0473506227,
0.1402883083,
0.2511122525,
-0.0766199082,
-0.8887055516,
0.1407387555,
-0.1085183918,
-0.2693585157,
0.1427569985,
0.0030162542,
-0.0376037844,
0.2874901891,
-0.0281252153,
-0.0584407821,
-0.3251951337,
-0.0211491734,
-0.5583201647,
0.4083808959,
-0.0712125078,
0.4945524931,
-0.1182284281,
0.6171674728,
0.20780918,
-0.0619169138,
0.2547045052,
0.0239901114,
0.6073728204,
-0.1072015464,
-0.2783245146,
-0.084859699,
-0.0239932146,
-0.0853274539,
-0.0090324134,
-0.0584643856,
0.1181460619,
0.0796117261,
0.2320279479,
0.0757559091,
-0.2434883714,
-0.0203367155,
-0.4235741198,
-0.0518676862,
-0.1387821883,
0.059023276,
0.2097692639,
-0.0455073975,
-0.0046002809,
0.4423108399,
0.3119581342,
0.0415234193,
-0.2268209159,
-0.1775471419,
-0.1501012743,
0.0896358266,
0.0007103857,
0.1634251028,
-0.2935024798,
-0.2467363179,
-0.0244638883,
0.2641925216,
0.1933015287,
-0.1021023616,
0.2468359023,
0.145140484,
-0.1282408684,
-0.2201013863,
-0.1688660085,
0.0715930462,
0.0720295832,
0.1230841056,
0.1096325964,
-0.0983215794,
-0.4640814364,
0.2271193862,
-0.1008078828,
-0.2255889922,
-0.2153090239,
-0.3647738397,
0.2151690871,
-0.155984655,
0.0056077479,
-0.0892066807,
-0.047691375,
-0.53020823,
-0.0354445949,
0.0433668569,
0.1319925487,
-0.0151181202,
-0.1747693568,
0.015892081,
0.3352846205,
0.0775938705,
0.312271446,
0.7089363933,
0.3806373179,
0.7758240104,
0.0514253713,
-0.0793999061,
0.0619845204,
-0.0522953458,
0.2830349803,
0.2635784149,
0.0025538418,
0.0171922985,
0.2338246554,
0.3215700388,
-0.505379796,
0.1569879651,
0.264218092,
0.1064850092,
-0.3957761824,
-0.2398792654,
0.5792211294,
0.1990605891,
-0.0721658766,
0.5011490583,
0.1718720794,
-0.1601869911,
-0.2222992331,
0.4092177749,
0.9316588044,
-0.0502673127,
0.1656300873,
0.0335484669,
-0.1023986638,
0.2151538581,
0.1712472439,
-0.2169052362,
-0.0240083244,
0.1363589615,
-0.0608063191,
-0.0942701772,
0.1299223453,
0.3960390091,
-0.1103717238,
0.1091582254,
-0.303781122,
0.3193358779,
0.0828708336,
0.0924633145,
-0.218165949,
-0.1045313776,
-0.2052214891,
0.0486940481,
0.0608363487,
0.1807583123,
-0.2541728914,
-0.0188480876,
0.446216017,
-0.1876959056,
-0.2039919496,
-0.1698860824,
-0.1652953327,
0.0798175037,
-0.0238747392,
-0.1340298653,
0.1769520193,
-0.0074081505,
0.1080762893,
0.1310154349,
-0.0535701551,
-0.0393222645,
-0.0277146921,
0.0349648669,
-0.3362423182,
0.0249800645,
0.2612010241,
-0.0432105549,
-0.2569174469,
0.0815665424,
-0.2410903573,
-0.4599913061,
-0.0117589468,
-0.0138682714,
-0.2203842103,
-0.2234914452,
0.1917606145,
0.2521336675,
-0.0509059206,
0.2003608793,
0.0938787535,
0.1300971806,
0.1086524352,
0.1496188939,
0.255274415,
-0.5631153584,
-0.2056601793,
0.3596984148,
0.1191543266,
-0.0834056437,
0.596660316,
-0.0755221397,
-0.0699011758,
-0.2034487873,
0.0813292116,
0.491258055,
-0.110657692,
-0.0511977375,
0.2134350538,
0.064825736,
0.1864901185,
0.0118828993,
0.3163927197,
0.0499115922,
-0.1891225427,
-0.2757257223,
-0.5968088508,
0.1763011217,
-0.5243194699,
0.1977214217,
-0.2581467628,
-0.15779607,
0.2630138099,
0.1634445339,
-0.2372754812,
-0.0650737584,
0.0795965493,
-0.006403524,
0.1266520917,
0.0493783616,
0.2707764804,
0.2368385941,
-0.0509185009,
-0.3025642037,
-0.4482941329,
-0.0827125162,
0.0779378489,
0.1980537772,
-0.0288227443,
-0.0505639389,
-0.2009483427,
-0.1481542438,
0.0085130613,
-0.0148984771,
0.3040984571,
0.1017025411,
0.0122185769,
0.2981624305,
-0.1341836601,
-0.0520498194,
-0.1450079829,
-0.1267636716,
0.1184034199,
0.1700136662,
-0.0528152362,
-0.1776983887,
0.0550749823,
-0.0531752557,
0.2609737515,
0.0785879865,
0.0470483638,
-0.1665111333,
0.168928653,
0.0177526455,
0.0832997933,
0.2856792212,
0.2641968131,
0.2026150227,
0.000116955,
-0.3949365914,
-0.1265986711,
0.1080260202,
-0.0788772851,
0.0302124955,
-0.2441766709,
0.4178747833,
-0.1300904304,
0.1016244292,
0.2072561532,
-0.0034331067,
0.5227724314,
0.1552022845,
0.2455792129,
-0.506616652,
0.2247365117,
0.2779004276,
-0.2484921962,
0.2828525305,
0.5526021719,
0.1590900272,
0.229198277,
0.2005200982,
0.1454209536,
0.5187020898,
0.3187766075,
0.0674998388,
-0.0710732564,
-0.5168736577,
0.0675183311,
0.6114203334,
-0.5252994299,
-0.1863863617,
0.0400246792,
0.1060191244,
0.1136127412,
-0.1601575017,
-0.2614753544,
-0.106664598,
-0.1605530381,
-0.1018734798,
-0.0088209212,
-0.0413443111,
-0.05631347,
0.0137309674,
0.0979529023,
-0.0557849407,
0.1776929498,
-0.2269347012,
-0.2977192104,
-0.1786247194,
0.0254455116,
0.2555102706,
0.1896307021,
-0.2225936949,
-0.0161185674,
0.2228962034,
-0.0998974964,
0.3993679881,
0.6683419347,
0.5541521907,
0.5690360665,
0.1320450306,
0.0655332133,
-0.0043255035,
0.0803078562,
-0.0206406433,
0.6196236014,
-0.0621435866,
-0.4615193307,
0.2826050222,
0.0600631535,
-0.0013747825,
0.3530262113,
-0.1989957392,
0.2381371856,
-0.0434098206,
0.1839701533,
-0.0043283757,
0.026796395,
0.0336169004,
0.1342734843,
-0.2274182737,
0.1501425952,
1.016428709,
-0.1379929185,
0.0296130795,
-0.326985091,
0.0603296608,
0.0394895785,
0.8118128181,
0.5566381216,
0.0179845206,
-0.2498926222,
-0.051355727,
-0.6797934771,
0.1075496301,
0.0582311302,
0.3735079169,
0.1561586857,
-0.1018715128,
0.0600337461,
0.2787947655,
0.1806214005,
-0.2345178425,
0.0183870848,
-0.4392371178,
-0.3589943647,
-0.0199515745,
0.3248647451,
0.2395901084,
-0.110691011,
-0.0422999486,
0.3283739686,
-0.0264968891,
-0.0363648236,
-0.0633308142,
0.2724046111,
-0.0000991305,
0.4668426812,
0.3117851615,
0.2629804015,
0.3411050737,
0.2029398829,
0.0029428091,
-0.4637704194,
-0.2727870941,
0.0260019824,
0.2566902637,
0.2327902019,
0.0843552724,
-0.084805496,
-0.0388636328,
-0.3371563554,
0.0666680932,
0.081819877,
0.0705151781,
0.079584308,
0.0076005282,
-0.0186115261,
-0.1575898677,
-0.0037081959,
0.1348816007,
0.4192293286,
0.1956193894,
-0.1185469553,
-0.3421120048,
0.3606152534,
-0.1771562248,
-0.0268313084,
-0.0998395309,
0.2550289333,
0.1801067889,
-0.2032318562,
-0.8239952922,
0.0944808125,
0.4521763325,
-0.1755989343,
-0.1675272286,
0.1317552179,
0.0573525466,
-0.0612022094,
0.1037154943,
0.4520232975,
-0.0139025208,
-0.1118363366,
-0.1715681702,
-0.4354243875
]
|
https://github.com/huggingface/datasets/issues/1942 | [experiment] missing default_experiment-1-0.arrow | Hi !
The cache at `~/.cache/huggingface/metrics` stores the users data for metrics computations (hence the arrow files).
However python modules (i.e. dataset scripts, metric scripts) are stored in `~/.cache/huggingface/modules/datasets_modules`.
In particular the metrics are cached in `~/.cache/huggingface/modules/datasets_modules/metrics/`
Feel free to take a look at your cache and let me know if you find any issue that would help explaining why you had an issue with `rouge` with no connection. I'm doing some tests on my side to try to reproduce the issue you have
| the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you. | 84 | [experiment] missing default_experiment-1-0.arrow
the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you.
Hi !
The cache at `~/.cache/huggingface/metrics` stores the users data for metrics computations (hence the arrow files).
However python modules (i.e. dataset scripts, metric scripts) are stored in `~/.cache/huggingface/modules/datasets_modules`.
In particular the metrics are cached in `~/.cache/huggingface/modules/datasets_modules/metrics/`
Feel free to take a look at your cache and let me know if you find any issue that would help explaining why you had an issue with `rouge` with no connection. I'm doing some tests on my side to try to reproduce the issue you have
| [
-0.035873726,
-0.0009465087,
0.0741713122,
0.1184611022,
0.0837400109,
-0.1097528413,
0.1720351577,
0.2317185253,
0.2638721466,
0.1391707808,
0.0678791031,
0.1733183414,
-0.2854747176,
-0.012178422,
0.1369280815,
0.0551353395,
-0.0628576949,
-0.0301498752,
-0.3259330392,
-0.1252782196,
-0.2200646102,
0.5307019353,
-0.0628680438,
0.0645004734,
-0.4562327862,
0.175228551,
-0.1284714937,
0.3620724678,
-0.083354868,
-0.5840671659,
0.3293272853,
0.1146460101,
0.0539535061,
0.4142382443,
-0.000119533,
-0.1037965491,
0.240008086,
-0.1888288409,
-0.2738631666,
-0.2778946161,
-0.0753618553,
-0.252202481,
0.5798792839,
-0.0966046676,
-0.1344477385,
0.091610916,
0.0100134742,
-0.4268663526,
0.4767813087,
0.1211742759,
0.1166025549,
0.4053640962,
0.0331891477,
-0.3333911896,
0.2213083953,
-0.1046054512,
0.0415685251,
0.4594832361,
0.0687562823,
0.0936743915,
-0.1464713812,
0.2114190608,
0.3470682502,
-0.184419781,
0.3098647296,
0.0112120146,
0.001315852,
-0.1081184149,
-0.0284115821,
0.3066343963,
0.2365592718,
-0.1305872798,
-0.2608055472,
0.1283789277,
-0.0879924521,
-0.4039273262,
0.1538643092,
-0.0939177498,
0.0763652623,
0.0891129449,
0.0873681456,
-0.1980053484,
-0.0160593428,
0.1474251449,
-0.1284568608,
-0.1398056298,
-0.1876241267,
0.0414876454,
-0.0495054126,
0.021950217,
-0.5056312084,
0.4271098971,
-0.1831524074,
0.1320417523,
-0.4020821452,
0.1370483041,
0.2792125642,
0.174356699,
0.0485687852,
0.5940816402,
0.0544067919,
0.097638458,
0.3240975738,
0.1152188554,
-0.1439854503,
0.463717103,
0.1471069306,
-0.0318708643,
0.229067862,
0.2400324792,
-0.0997859836,
-0.2906395793,
0.2207399607,
-0.2854763269,
0.18021065,
0.2259310484,
0.2352600694,
-0.3656279147,
0.010947275,
0.2513976693,
-0.1731030345,
-0.0880763829,
0.0778520107,
0.3774323165,
0.0209160019,
-0.0662797838,
0.3336496055,
0.3538492918,
-0.2321901321,
-0.0483001024,
-0.4542720318,
-0.1579868644,
-0.1675678641,
0.3084726632,
0.1213163659,
-0.0429606922,
0.5225878954,
-0.1377248019,
0.3675762713,
-0.0634159371,
0.297954917,
0.149572432,
-0.0578732751,
0.2460674793,
0.0045490703,
0.1730863452,
0.3100201488,
-0.0152879106,
-0.1046917811,
-0.3136593401,
-0.2143966407,
-0.4901869297,
0.0863236934,
0.0288707744,
-0.3260271549,
0.184158355,
0.3849879503,
0.0545696206,
-0.2149498463,
-0.258146584,
0.1204286665,
0.0444046222,
-0.0732084364,
-0.1320746988,
0.5114515424,
0.7114234567,
-0.2573742568,
-0.4081453383,
0.2153408527,
0.0144806439,
-0.1083218679,
0.1975732893,
-0.0630954206,
0.1563925296,
-0.3403096199,
-0.104398042,
0.4113847911,
-0.5171523094,
-0.4667465687,
-0.0168592501,
-0.3854105473,
-0.1010333374,
0.100802727,
-0.0357387289,
0.0745940059,
0.2175189108,
0.1196309105,
-0.1724983454,
0.2414471954,
-0.3219717741,
-0.2602000535,
-0.205573082,
0.1193033457,
-0.0603428483,
0.2362510413,
0.1523996592,
-0.0052175093,
-0.0732077062,
0.1255849004,
0.1020375639,
0.198178187,
0.3781461716,
0.2921811342,
0.1836387664,
0.1978510171,
0.1566253752,
-0.1678198874,
0.2583169341,
-0.4689187407,
0.1527777761,
-0.050325077,
-0.0147544388,
-0.2192660868,
-0.2279685438,
-0.0850846842,
-0.4767321348,
0.0177704878,
0.0121324342,
0.2931830585,
0.3102687299,
-0.1623553187,
0.271412909,
0.0924600214,
0.2920162976,
-0.665667057,
0.0322583206,
-0.111371994,
-0.3356736302,
-0.0986843705,
0.1647351235,
0.0611553863,
-0.0081723323,
-0.1790617555,
0.388335377,
-0.0169806294,
0.1749453098,
0.3794829845,
0.0891622901,
-0.0574565567,
-0.4079512656,
-0.0328812003,
0.1797970533,
0.054246597,
-0.0016228952,
-0.200785026,
0.323792249,
-0.0825715661,
0.1331495494,
-0.2450240403,
0.3666040003,
-0.0399597734,
-0.068823278,
-0.5726245642,
-0.0933301747,
0.3485017717,
-0.1906698644,
0.4904284775,
-0.1709934324,
-0.0320259966,
0.0218921006,
0.0991404131,
0.1401264369,
0.2501839101,
-0.0037822735,
-0.2171487957,
-0.0424430557,
0.0269455593,
-0.145960629,
0.435215354,
0.1362624615,
0.1439884007,
0.1243443638,
0.2096871585,
-0.1694743633,
0.042471692,
-0.0767041147,
-0.1214769706,
0.0951079726,
0.1849321425,
0.1680720896,
-0.2335235029,
-0.1956409812,
-0.3971342742,
0.0322262943,
-0.3484333158,
0.0946543217,
-0.2037185133,
0.3463998139,
0.3030624986,
-0.0559964627,
-0.3411485553,
-0.2638902068,
0.1385769695,
-0.1877670586,
0.0301665831,
0.2229872644,
-0.2555915713,
0.4870508015,
0.135251075,
-0.1863756925,
-0.3071425259,
-0.2004611343,
-0.1395706087,
-0.1502984315,
-0.2870167494,
0.0871473774,
0.0249529425,
-0.2135682702,
0.1292313635,
-0.0343738869,
-0.3483103514,
0.0483235791,
0.0518325493,
0.5172299743,
0.3936597407,
0.0021314784,
-0.2833369374,
0.2200522721,
0.5371550918,
-0.3732430339,
-0.3090991676,
0.0990039185,
0.058336556,
0.2021282464,
-0.2552038729,
-0.3111088574,
-0.0462788865,
-0.3856251836,
0.6103230119,
0.1012191847,
-0.1091394797,
-0.0645339116,
0.0123225143,
0.1742913574,
-0.2101200521,
0.36278072,
-0.3324127197,
-0.649390161,
0.3374322653,
-0.1905548871,
-0.3684260547,
0.0284810793,
0.0337614901,
0.3815255761,
-0.0200922545,
-0.5352696776,
-0.3894003332,
0.0445667095,
0.2492182702,
-0.1060671434,
0.0623193569,
0.5808557272,
-0.0551422052,
-0.0207334105,
-0.058793731,
-0.1301968098,
0.4343072176,
-0.1079046354,
0.1379552931,
-0.101251021,
0.4365176558,
0.0528163686,
0.7605791688,
0.4823164344,
0.2873231471,
0.2661586404,
0.0358390957,
0.4865822792,
-0.2143175751,
-0.1602760404,
0.1947720498,
0.194983393,
-0.0684700012,
0.1258611381,
0.1112141237,
0.2847435474,
-0.2191252559,
-0.0268843099,
-0.3354534507,
-0.3300554752,
-0.020689277,
-0.2873110175,
0.2177422196,
-0.0690014586,
0.0413904339,
-0.2042779475,
0.0539592914,
0.3671606779,
0.3646480739,
0.2758911252,
0.2595492303,
-0.2775644958,
0.0905445591,
-0.586440146,
0.317091167,
-0.5184069872,
0.1670906246,
-0.2591428161,
-0.2926996946,
0.0993681997,
-0.0163173098,
0.5642927289,
0.0010579024,
-0.170298025,
0.0686849058,
-0.260720998,
-0.5911591053,
-0.0195033178,
-0.0470784679,
-0.0120290602,
0.2525357008,
0.2193754017,
-0.3621322811,
-0.1756045371,
-0.1045859531,
-0.1455085725,
-0.2161612511,
-0.1501789242,
-0.3854458332,
0.032044813,
-0.1962871403,
0.1033233851,
-0.2960125804,
0.0171898417,
0.0963181034,
0.0973910391,
-0.2283383608,
-0.0517569706,
-0.0071312794,
0.0747465938,
0.3057209849,
-0.3038362563,
0.1706120074,
0.4674375653,
0.0272761639,
-0.0002300525,
0.5265595317,
0.0551017895,
-0.2797265053,
0.2987195253,
-0.063192673,
0.2123973072,
0.5377956033,
-0.2559634447,
0.1114688441,
-0.0735082403,
0.3654130697,
-0.1908655167,
-0.1126983613,
0.3272385597,
-0.1773874164,
0.3741320372,
0.1637036502,
0.0672194287,
0.158640787,
-0.138021633,
0.1574548185,
0.0404476784,
-0.1430934072,
0.3999296725,
-0.1042415202,
0.9887389541,
0.2283458859,
0.2489137203,
0.1314040869,
0.0513561219,
0.454508841,
0.0168126337,
0.022108702,
-0.2404447645,
-0.2034012526,
-0.0113505498,
-0.1185374036,
0.0253494121,
-0.2338591814,
-0.2589438558,
0.414023608,
-0.3903064132,
-0.05178703,
-0.2063386738,
-0.1757091433,
-0.2596441805,
-0.116449587,
0.012173444,
0.0868628472,
0.0721113756,
0.6109396815,
-0.2037318945,
-0.1589173675,
-0.4563349187,
-0.3336652815,
-0.0674574599,
0.013048443,
-0.3301525116,
0.0881634429,
0.2974458635,
-0.1824515611,
-0.1842075884,
0.3558036387,
0.3632246554,
0.1726472974,
-0.2810918093,
0.0607844889,
0.0395959467,
0.0463075042,
0.1197912693,
0.0193878636,
0.2255720496,
0.0099451868,
-0.1676050574,
0.164151147,
-0.2100360394,
0.0512364991,
0.0139882388,
-0.0534160398,
-0.1955827326,
-0.113757506,
-0.020223191,
0.1057810858,
0.0967819095,
-0.1549050212,
0.0860569254,
0.3396831751,
-0.1765408069,
-0.0151250092,
0.168272227,
-0.204136312,
-0.0545962751,
0.5798854232,
-0.3019779921,
-0.1088525727,
0.3987403512,
0.0334340148,
-0.0022549634,
-0.1496373117,
0.1192859709,
0.4620960653,
-0.7651281953,
0.0641342029,
0.0818872303,
0.0467189029,
0.0998904929,
0.1696161479,
0.3279044032,
-0.1738366038,
0.0599946864,
-0.2481648922,
-0.4355519414,
0.3564049304,
-0.1347400546,
0.1367653757,
-0.3409822881,
-0.087152943,
-0.0929879844,
0.2171244174,
-0.2840718925,
0.1434051841,
-0.283287704,
-0.1181835011,
-0.0723362938,
-0.1272995621,
0.3977063894,
-0.0696900412,
-0.0448788367,
0.0667281523,
-0.4174090922,
-0.1143615693,
-0.1739068925,
0.1288141459,
-0.134773314,
0.1333722174,
-0.0616761483,
0.2145867348,
0.0732684731,
-0.1694598347,
0.1197838932,
0.217256248,
0.0872650519,
0.072912775,
-0.0300871637,
0.0965832695,
-0.1226503327,
0.2455373406,
0.1651062965,
-0.1488166749,
-0.07610102,
0.020768417,
-0.3127218783,
-0.0915165395,
0.1688802391,
0.1058796868,
-0.1790584624,
0.1402074546,
0.1360842735,
-0.0907969773,
-0.3047739565,
-0.218834877,
0.400414139,
0.1239717528,
-0.178718552,
0.0696372241,
-0.0565709472,
0.1327505559,
-0.0852432773,
0.3196440041,
0.0953549445,
0.339517206,
0.1357049644,
0.2389128208,
0.1446432173,
-0.0525929779,
0.0786917806,
0.1679390669,
-0.0646351427,
-0.1023807377,
0.3450416028,
-0.1604188383,
0.0050427322,
0.2077208012,
0.5121742487,
0.176421836,
0.0708959103,
0.0435598269,
-0.0611320399,
0.2262658626,
-0.1423234046,
0.2956942916,
-0.1136341318,
-0.2759341896,
-0.1424784362,
0.031171253,
-0.0090722069,
-0.2327332497,
-0.4316948056,
0.7024185658,
-0.2806924284,
-0.3212978244,
-0.1667736024,
0.1408961266,
-0.1320925355,
-0.2029647678,
-0.1144989282,
-0.139298439,
-0.1908464134,
-0.1848255098,
0.2290683836,
-0.0132927643,
0.0634704828,
0.1439852864,
0.0835377648,
-0.2804713845,
-0.4855870605,
0.1773282886,
0.1425659508,
-0.0415459201,
0.0629003718,
0.1436044574,
-0.1707990021,
0.1758074015,
0.6104491949,
0.2904424369,
0.0435972176,
-0.1393886507,
0.2393371463,
0.2593467236,
-0.0496915691,
-0.1322290152,
0.1564970762,
0.2695862949,
-0.4744096696,
0.0466503426,
0.0854166523,
-0.1447211504,
0.3480510712,
-0.3163051903,
0.3591749668,
-0.153848663,
0.3500989974,
-0.2364436388,
0.1475693434,
-0.1308509409,
-0.1009434909,
-0.7179360986,
0.1895139068,
-0.1232710183,
0.0928877071,
0.0719828084,
-0.3484202623,
0.0471335948,
-0.1072721183,
0.2144944817,
0.430064559,
0.162947759,
-0.2852115631,
-0.3108714819,
-0.3476510048,
0.2463074923,
0.07309746,
0.2230473161,
-0.1770626605,
0.4440940022,
-0.1031214595,
0.0922273248,
0.2410823256,
0.1612482816,
-0.0969932005,
0.0524555035,
-0.4813615084,
0.166090101,
-0.1789523661,
-0.0562410504,
0.0814187452,
0.2535747588,
-0.1228971332,
-0.1291747242,
0.0204992108,
0.1910952777,
-0.2418769896,
-0.2137033343,
0.1613464653,
0.5954048634,
0.172268033,
0.1285200715,
0.068503499,
-0.1416750252,
-0.308862716,
-0.2423846424,
0.0062137735,
0.087111257,
-0.1713272929,
0.3439911306,
-0.2581761479,
-0.4227479398,
-0.3795553148,
0.3892645538,
0.1324902326,
-0.1534836292,
-0.118877992,
0.3110212386,
-0.0710717812,
0.0651842952,
0.1813979447,
0.2737932801,
-0.0974208713,
0.332916826,
-0.3157680631,
-0.197163552,
0.6771653891,
-0.3671155572,
0.280562371,
-0.26948452,
0.5114611983,
0.3368268013,
-0.3409712017,
-0.3263528943,
0.1121217981,
0.0807696506,
0.0419808552,
-0.1084787324,
0.1534227282,
-0.3455203474,
0.03237205,
-0.1215449348,
0.3072327077,
0.1546481103,
0.1746725887,
0.0677531138,
-0.1550118923
]
|
https://github.com/huggingface/datasets/issues/1942 | [experiment] missing default_experiment-1-0.arrow | Thank you for clarifying that the metrics files are to be found elsewhere, @lhoestq
> The cache at ~/.cache/huggingface/metrics stores the users data for metrics computations (hence the arrow files).
could it be renamed to reflect that? otherwise it misleadingly suggests that it's the metrics. Perhaps `~/.cache/huggingface/metrics-user-data`?
And there are so many `.lock` files w/o corresponding files under `~/.cache/huggingface/metrics/`. Why are they there?
for example after I wipe out the dir completely and do one training I end up with:
```
~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock
```
what is that lock file locking when nothing is running? | the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you. | 93 | [experiment] missing default_experiment-1-0.arrow
the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you.
Thank you for clarifying that the metrics files are to be found elsewhere, @lhoestq
> The cache at ~/.cache/huggingface/metrics stores the users data for metrics computations (hence the arrow files).
could it be renamed to reflect that? otherwise it misleadingly suggests that it's the metrics. Perhaps `~/.cache/huggingface/metrics-user-data`?
And there are so many `.lock` files w/o corresponding files under `~/.cache/huggingface/metrics/`. Why are they there?
for example after I wipe out the dir completely and do one training I end up with:
```
~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock
```
what is that lock file locking when nothing is running? | [
0.2474490553,
0.0509266518,
0.0548085123,
0.2140232921,
0.1152905971,
0.1876895577,
0.227650851,
0.3381587565,
0.2461999357,
0.1004049554,
0.1633259058,
0.1200303733,
-0.3513060212,
-0.1723564416,
0.0993689373,
-0.027356524,
0.1081290171,
-0.0378127284,
-0.2317158431,
-0.164974466,
-0.2525987625,
0.5168856978,
-0.0808427259,
0.0938806832,
-0.564119935,
0.2315037698,
-0.160138905,
0.2439413965,
-0.0434487425,
-0.5871254802,
0.3680898845,
0.1198715493,
-0.0636296496,
0.5067222118,
-0.0001260311,
-0.1185864955,
0.2515879571,
-0.1634839624,
-0.2404904813,
-0.1307277381,
-0.0129827512,
-0.2789449394,
0.4915620685,
-0.2036508024,
0.1352378875,
-0.0842317045,
0.121447064,
-0.4259886742,
0.351277113,
0.0431350395,
0.0366242267,
0.2477324009,
-0.1989924163,
-0.2882103324,
0.1527168751,
-0.0924046636,
0.0252721012,
0.3664673269,
-0.0836110711,
-0.0094821826,
-0.2560974061,
0.3051269352,
0.2019365877,
-0.1374052912,
0.3521672785,
0.0150465863,
-0.0426554233,
-0.2301337719,
0.0013808173,
0.2264510095,
0.2374702394,
-0.1862585694,
-0.2228963971,
-0.0533671379,
-0.0899374709,
-0.2590973675,
0.2228355557,
-0.052190572,
0.0696588904,
0.1424811482,
0.0115559017,
-0.197601378,
0.0148123009,
-0.0037732827,
-0.1053487659,
-0.005746007,
-0.1575380713,
-0.015248538,
-0.0514958613,
0.1989749819,
-0.3450111449,
0.3006658852,
-0.0482684188,
0.2071721703,
-0.2239118069,
0.0468745269,
0.19790034,
0.11995285,
0.0460015349,
0.427505821,
0.022846425,
0.026350515,
0.4165909588,
0.0909777284,
-0.1048304811,
0.4695405066,
0.3464440107,
-0.067351304,
0.0851759166,
0.1602268368,
-0.1593468636,
-0.2561874092,
0.1269485056,
-0.2231048942,
0.2775917053,
0.0905746371,
0.0148455054,
-0.4099764526,
0.0961337686,
0.3147747219,
-0.2490133792,
-0.2345288843,
0.0893957093,
0.3153007925,
0.2518268526,
-0.0041998359,
0.180839628,
0.2091760635,
-0.2426619232,
-0.0500774346,
-0.3978199661,
-0.1054065824,
-0.2283215076,
0.350231111,
0.0507633835,
-0.1394726038,
0.7004557848,
-0.2605068982,
0.3893994689,
-0.1397275627,
0.2826370597,
0.2533381879,
0.0007689086,
0.4032490253,
-0.0881249681,
0.2115937471,
0.3516605794,
0.0514885075,
-0.1892666072,
-0.2525212467,
-0.2373770773,
-0.518161118,
0.0860112309,
-0.0284102019,
-0.5039557815,
0.2390987873,
0.4408908188,
0.147569418,
-0.0292730033,
-0.1374206841,
0.2848836482,
0.0987272263,
0.0174056124,
-0.1247297674,
0.3923793733,
0.7586100698,
-0.2284235209,
-0.4756801426,
0.1044254974,
-0.0117907496,
-0.0543394126,
0.14773269,
-0.0291077457,
0.2321293056,
-0.2840010524,
0.1421151906,
0.3668583035,
-0.3609600067,
-0.3620804846,
-0.010619225,
-0.3919944465,
-0.1379084736,
0.182104364,
0.0160974022,
0.0728414878,
0.1438128948,
0.1258191615,
-0.3850254714,
0.1877111495,
-0.4215300381,
-0.3467740715,
-0.1896636188,
-0.0304801147,
-0.1046682596,
0.2787399888,
0.0370404199,
0.0111546209,
0.1009097397,
0.1036302596,
0.1744165272,
0.1748632193,
0.2626433671,
0.2613891363,
0.1620638371,
0.2222009599,
0.193080917,
-0.3110492527,
0.2377901226,
-0.3628160357,
-0.0176316518,
0.110218659,
-0.0732134581,
-0.1398179978,
-0.235202983,
-0.1244362667,
-0.5270229578,
-0.0462954268,
-0.0360658728,
0.1183854565,
0.1227986217,
-0.2726367712,
0.147312656,
-0.0587888397,
0.3274552226,
-0.5532154441,
0.0320396088,
0.027866222,
-0.1970098168,
-0.2257990092,
0.1093444228,
-0.0025991036,
0.0232681092,
-0.0975058451,
0.380703032,
-0.0499366149,
0.183206141,
0.5586857796,
0.3541170955,
-0.1420737952,
-0.3481734693,
0.0158952605,
0.0016874633,
-0.1003442481,
-0.0012895826,
-0.2387944162,
0.3765333295,
0.1348475814,
0.1348783672,
-0.3614093959,
0.2761766613,
-0.2093581855,
-0.1233221218,
-0.5444703698,
-0.1353486925,
0.4068961143,
-0.2476065308,
0.3068442047,
-0.0123207457,
0.002422259,
0.0304552633,
0.1419316083,
0.04834418,
0.0429758616,
0.0081626298,
-0.2377899289,
-0.0533850156,
-0.0278110467,
0.0037658862,
0.4933542907,
0.1662804186,
0.2230533063,
0.0144518046,
0.1677997261,
-0.2444669455,
0.0901629329,
0.17396909,
-0.2210898101,
0.1015082449,
0.1378707439,
0.2083471864,
-0.294652164,
-0.0503391102,
-0.234801501,
0.031876985,
-0.3016398251,
0.1512338072,
-0.1539790481,
0.3830915689,
0.0986963734,
-0.0210699365,
-0.2364773452,
-0.3332991004,
0.2775133252,
-0.3183351457,
-0.027029017,
0.2005963773,
-0.3842664361,
0.6387848258,
0.0182665624,
0.0167177003,
-0.2889690101,
-0.0855257735,
0.0128289405,
-0.2142505497,
-0.1412097663,
0.0268614143,
0.0817524269,
-0.2668958604,
0.0615632161,
-0.1273289174,
-0.495573163,
0.0512228459,
-0.0161663108,
0.6132106185,
0.282838583,
0.0391496941,
-0.3447294235,
0.0620120168,
0.2875091136,
-0.2682443261,
-0.3798855543,
0.0450981706,
0.0512686111,
0.2367150933,
-0.3826584518,
-0.3334691525,
0.1506502926,
-0.3048088551,
0.579578042,
-0.0784144923,
-0.0105532818,
-0.123632662,
-0.1348556727,
0.2229507267,
-0.3493738472,
0.2714141309,
-0.3231590986,
-0.78293401,
0.4090440869,
-0.0861364827,
-0.04923914,
0.0389751829,
0.0890198648,
0.3141538203,
-0.1025537625,
-0.7185191512,
-0.4109578431,
0.0587696396,
0.183712557,
-0.0423480868,
0.0645670891,
0.4620447457,
-0.0869354457,
-0.0113906255,
-0.1259305924,
-0.1665929854,
0.4881490171,
-0.1238274202,
0.1869423985,
-0.1846829802,
0.3211716115,
0.1527523547,
1.0194028616,
0.5859873891,
0.353109628,
0.136278525,
0.2488947511,
0.4330226183,
-0.1152088642,
-0.0847559273,
0.2251206338,
0.2476469725,
-0.1349828243,
0.3065978885,
0.2231493145,
0.1870115697,
-0.0333175622,
0.0093362331,
-0.317751646,
-0.2941359282,
-0.0049865786,
-0.1403052211,
0.227934286,
-0.0769745559,
0.1744734496,
-0.1848290563,
0.0769241825,
0.3933353126,
0.58130759,
0.3059709966,
0.2613006234,
-0.1821736395,
-0.1309936643,
-0.5380689502,
0.1992972791,
-0.4458006918,
0.0942807198,
-0.2295227647,
-0.3128387034,
0.2112235576,
0.1381263584,
0.5694952607,
0.1833450049,
-0.220147416,
0.0576130152,
-0.1568569988,
-0.2315967232,
-0.1577764601,
-0.004672443,
0.0672968701,
0.2795844674,
0.394103229,
-0.390599966,
-0.3081786335,
-0.1242816374,
-0.1054900363,
-0.3238488138,
-0.2797121108,
-0.2802342176,
0.0235747825,
-0.0901427791,
0.0684608519,
-0.2081900239,
-0.0828770176,
0.0170267336,
0.1113158241,
-0.0379927494,
-0.087601006,
0.1582573801,
0.074530676,
0.2948907018,
-0.2337712646,
0.1438095123,
0.5626403093,
-0.0551483929,
0.0503554977,
0.4550113678,
-0.024470346,
-0.0061210725,
0.3603447676,
-0.1528995335,
0.3670767248,
0.6862483025,
-0.1970186085,
0.1456226557,
0.0650582612,
0.3557296991,
-0.0740637258,
-0.0981432199,
0.3586218953,
-0.1224852428,
0.3750060201,
0.1454331428,
0.2473687083,
0.2430826128,
-0.1910924911,
0.3061974347,
0.1086223796,
-0.1157655418,
0.3354496658,
0.0150601501,
1.0408426523,
0.2446960062,
0.2499575764,
0.0714623779,
0.131248787,
0.3983027637,
0.064664565,
0.0467553101,
-0.1198776364,
-0.1944518983,
-0.0132672535,
-0.1940736622,
-0.0282719322,
-0.2923018038,
-0.1856505722,
0.4099218547,
-0.4929341078,
-0.1550749838,
-0.1195692271,
-0.3021264076,
-0.2614346445,
-0.0750588998,
0.1547849923,
-0.0412604548,
0.129280895,
0.5553104281,
-0.2514208257,
-0.2232624292,
-0.3335106075,
-0.2589261532,
-0.1489759535,
-0.2111895531,
-0.189193666,
-0.0236805342,
0.3486908376,
-0.1595159322,
-0.151300922,
0.2677079141,
0.3971246183,
0.0755351111,
-0.3020040989,
0.0638312399,
0.168032527,
0.0085618645,
0.0653496757,
0.0340458304,
0.3502118289,
0.0003314781,
0.051117979,
0.0249728505,
-0.3353210092,
0.0492203161,
-0.0794572234,
-0.0835643187,
-0.0526295081,
-0.0755613893,
0.0267641246,
0.2042146176,
-0.0968355685,
-0.1874866039,
0.0331465676,
0.3781020343,
-0.1960802972,
-0.0299339723,
0.1211582348,
-0.3434658945,
-0.056023214,
0.4342232645,
-0.1925753057,
-0.1195446551,
0.5066856146,
-0.0645437092,
-0.1223666221,
-0.0741557032,
0.1144796684,
0.4430144429,
-0.7368451357,
0.0767195448,
0.0242283251,
0.027922295,
0.076529637,
-0.0071542314,
0.5015127063,
-0.1989101022,
-0.2411553711,
-0.2157037556,
-0.3882726431,
0.382838726,
-0.304048717,
0.132484585,
-0.6194870472,
0.059451852,
-0.0539758243,
0.1296787411,
-0.2124765962,
0.2109170705,
-0.2437582314,
-0.1170597598,
-0.0746393353,
0.034662161,
0.291379869,
-0.0565142073,
-0.1333196014,
0.0286499355,
-0.3847767413,
-0.0517192371,
-0.1915146858,
0.1899384558,
-0.1081531867,
0.2442278713,
0.0066735838,
0.3041705489,
0.0198464151,
-0.0583771951,
0.0101107797,
0.2571415007,
0.1279611439,
0.1851986647,
0.1432300508,
0.2480726242,
-0.0729073435,
0.2049414217,
0.1763908118,
-0.1000492349,
-0.100021638,
-0.103972584,
-0.4285862744,
-0.0848950073,
0.1566680372,
0.1853018701,
-0.3170949519,
0.0999708399,
0.0618540645,
0.017347144,
-0.2353607416,
0.0403750166,
0.2111481577,
0.2245329767,
-0.2050517797,
0.0644704774,
-0.0084530739,
0.0521703064,
0.0229846183,
0.2303783298,
-0.0552914217,
0.4240315259,
-0.0268420093,
0.2237038016,
0.1670221835,
-0.1279321164,
0.1427687854,
0.1989018619,
-0.0372639485,
-0.0433575921,
0.3380185068,
-0.0348776877,
0.1034381762,
0.3432769477,
0.5569768548,
0.1540989727,
0.0934894234,
-0.095464997,
0.0071916617,
0.2507783771,
-0.0900276452,
0.2582259476,
-0.2793653011,
-0.1373145729,
-0.2210548073,
0.1737849712,
0.0515685976,
-0.1402486414,
-0.3882139623,
0.8121158481,
-0.1609298885,
-0.2608855665,
-0.0700841695,
0.1249647811,
-0.1619194597,
-0.1566227078,
-0.1197467595,
-0.1842711866,
-0.1253314912,
-0.1607532799,
0.2283630222,
-0.0062960899,
0.142766729,
0.0652465373,
-0.0398544557,
-0.1074963883,
-0.4368210733,
0.2725353539,
0.2284075469,
-0.2035956979,
0.0297516007,
0.1274763793,
-0.2528051138,
0.2495365739,
0.5566775203,
0.2099298835,
-0.0059628258,
-0.116363585,
-0.0035183749,
0.2883097231,
-0.0202124286,
-0.046643246,
0.2402109802,
0.1830726862,
-0.6153120995,
-0.0621646941,
0.0086089661,
-0.1076652482,
0.388800621,
-0.2084247768,
0.3229672611,
-0.1921548992,
0.3530452847,
-0.2691182792,
0.0926730484,
0.0585445054,
-0.0918288678,
-0.7468355298,
0.2137381881,
-0.1359360665,
0.0162217021,
0.0330401994,
-0.2474637479,
0.0152216805,
-0.095531553,
0.1805480123,
0.5530499816,
0.0532684363,
-0.3335348964,
-0.3067728281,
-0.4826698899,
0.2709023356,
0.1446659416,
0.4335973859,
-0.0228388999,
0.3380470872,
-0.0630273893,
-0.0161663722,
0.2461424023,
-0.0855208337,
-0.1611034125,
0.004001298,
-0.5652292371,
0.1719990671,
0.0195853617,
-0.0025385092,
0.1001019776,
0.2926966548,
0.0207595024,
-0.2334727347,
-0.0914474577,
0.2584429979,
-0.211258918,
-0.055337809,
0.3531957567,
0.4249584377,
0.0721088797,
0.0359959267,
0.0304969978,
-0.199416548,
-0.2580091357,
-0.1420318633,
-0.0411229506,
-0.1456899196,
-0.2933090627,
0.3412894607,
-0.4688860178,
-0.4392543733,
-0.273901999,
0.2539842427,
0.0947204679,
-0.1432621479,
-0.3065035939,
0.3340898752,
-0.2120443135,
0.0159965567,
0.3520704806,
0.3827581406,
0.0340381563,
0.2756769955,
-0.3647692502,
-0.1141462773,
0.7994847298,
-0.554184556,
0.2426100522,
-0.192760095,
0.3955247104,
0.5046322346,
-0.3508095443,
-0.4224115014,
-0.0967805088,
0.1382527798,
0.0550412834,
-0.2188418806,
0.1441937983,
-0.4663902223,
-0.0743133575,
-0.0288975872,
0.2651973367,
0.1610632986,
0.054708764,
0.0375732817,
-0.1937300563
]
|
https://github.com/huggingface/datasets/issues/1942 | [experiment] missing default_experiment-1-0.arrow | The lock files come from an issue with filelock (see comment in the code [here](https://github.com/benediktschmitt/py-filelock/blob/master/filelock.py#L394-L398)). Basically on unix there're always .lock files left behind. I haven't dove into this issue | the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you. | 30 | [experiment] missing default_experiment-1-0.arrow
the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you.
The lock files come from an issue with filelock (see comment in the code [here](https://github.com/benediktschmitt/py-filelock/blob/master/filelock.py#L394-L398)). Basically on unix there're always .lock files left behind. I haven't dove into this issue | [
0.0978653282,
0.0017493968,
0.1070124134,
0.1418966502,
-0.0015059871,
0.0359288268,
0.2448604107,
0.2713265419,
0.2091091424,
0.1213854998,
0.1688696742,
0.1361202896,
-0.3981263936,
-0.0413281024,
0.0657580122,
0.0775554329,
0.0212285146,
-0.0395922288,
-0.316653043,
-0.1807793826,
-0.2565912604,
0.5491790771,
-0.1192008257,
0.0093759969,
-0.5109117627,
0.2307160199,
-0.2306174487,
0.2772123516,
-0.0274778139,
-0.5815619826,
0.389434278,
0.1215362474,
0.0478555262,
0.4788637459,
-0.0001274045,
-0.1663456857,
0.1572808772,
-0.186996147,
-0.1530370265,
-0.1362830549,
0.0305326656,
-0.296793431,
0.5233108401,
-0.2339411527,
0.0798513144,
-0.0288460795,
0.1023539528,
-0.2997953892,
0.4383219182,
0.0624675117,
0.0467605293,
0.3075241745,
-0.0342119299,
-0.2570322752,
0.0702648684,
-0.0924274549,
0.0015269269,
0.3444685936,
-0.2050894797,
0.092100203,
-0.2054679692,
0.2364491969,
0.2473952025,
-0.1546231806,
0.2657469213,
0.0201909039,
-0.0640987083,
-0.1387816817,
-0.0949210376,
0.3408108056,
0.1928485483,
-0.1521594971,
-0.2342790216,
0.0592426173,
-0.1122281626,
-0.364672482,
0.2042402327,
-0.070137918,
0.1202767119,
0.1426340342,
0.0680516735,
-0.1823937893,
-0.0471959114,
0.0707627535,
-0.1521102041,
0.0407566056,
-0.1404024512,
0.0206549056,
-0.0408882834,
0.1827444583,
-0.4113307297,
0.3544475734,
0.0115480414,
0.1267995387,
-0.3444469273,
0.1168116555,
0.2434756309,
0.0282621346,
0.0463361405,
0.405618459,
-0.0580374002,
0.0199385192,
0.39591223,
0.1504535824,
-0.0784413368,
0.4660256207,
0.2361449748,
0.0168705694,
0.1764597744,
0.1849359721,
-0.2115629315,
-0.2638480067,
0.1365804523,
-0.2362144887,
0.4281723499,
0.1122878045,
0.1105808467,
-0.4319954813,
0.162894547,
0.2799921632,
-0.1437160075,
-0.1873988062,
0.096094504,
0.3751056194,
0.1744754314,
0.0033349565,
0.2302556932,
0.3915508986,
-0.2153753936,
0.0076533086,
-0.3904956579,
-0.2105622739,
-0.0540186092,
0.3026126623,
0.1356307268,
-0.1125970334,
0.6726794839,
-0.2044870257,
0.4261625707,
-0.090132758,
0.328532815,
0.2119804025,
0.0645420253,
0.3416905999,
-0.0264649056,
0.2123864889,
0.2896437347,
0.1480875313,
-0.2393878251,
-0.2758549154,
-0.1812230945,
-0.5380880237,
0.0798787102,
-0.0424434766,
-0.4659704864,
0.1792512685,
0.4528219104,
0.146415323,
-0.1015214771,
-0.3035160005,
0.2009051591,
-0.0098101413,
-0.0084781516,
-0.1502696425,
0.5102131963,
0.6958428621,
-0.3465330899,
-0.3804933429,
0.0367822275,
0.0076957485,
-0.005418777,
0.261105746,
0.0363230109,
0.1693779826,
-0.3158248961,
0.1305025518,
0.3877379,
-0.3373552561,
-0.45686993,
-0.0679740459,
-0.3683250844,
-0.1110467389,
0.192932263,
0.013398923,
0.1307076365,
0.1547204256,
0.0300891586,
-0.2190194577,
0.2606107593,
-0.3739932775,
-0.3569114208,
-0.2306002527,
0.0067295413,
-0.092092447,
0.3358698785,
0.0176149886,
0.0747952759,
0.0229977872,
0.0826774612,
0.075203225,
0.1626363397,
0.2784368098,
0.3372357786,
0.1000658572,
0.2661376595,
0.1485666931,
-0.209230125,
0.2349853814,
-0.4146917462,
0.0708449781,
0.1174755543,
0.0061448957,
-0.1154767349,
-0.2034731358,
-0.0905113667,
-0.4177536964,
-0.0547222868,
-0.0495189875,
0.2109535635,
0.2493547797,
-0.1767959446,
0.0389821976,
0.0869968832,
0.2594942153,
-0.4751547575,
0.0103414906,
-0.0947172791,
-0.3357906342,
-0.0653162897,
0.1485405266,
0.0108670918,
0.0354322419,
-0.1438515782,
0.3710698187,
-0.1724668592,
0.2730035186,
0.4506233931,
0.2415378988,
-0.1196840927,
-0.3655082285,
0.0325868055,
0.142440632,
-0.1088574156,
0.0363417193,
-0.245175004,
0.382999748,
0.2120265067,
0.0713483766,
-0.3359494209,
0.3146032393,
-0.0740355924,
-0.0987766907,
-0.4815728068,
-0.1280821115,
0.3917380273,
-0.2381819636,
0.241021961,
-0.1055559516,
0.0105513772,
0.0025618025,
0.2497160286,
0.0799552202,
0.233252719,
0.0780289024,
-0.2227702439,
-0.0949149057,
0.0184848923,
-0.1300746948,
0.4450001419,
0.1906783432,
0.2278555036,
0.0538470522,
0.1052548811,
-0.1888126582,
0.1054110453,
-0.0117074195,
-0.1605948359,
0.1285530925,
0.2171353847,
0.2108033895,
-0.2892756164,
-0.1394120306,
-0.4038007259,
-0.0391596816,
-0.3721092343,
0.0799336955,
-0.2586636543,
0.4104160964,
0.1836584359,
0.1734473556,
-0.2760926187,
-0.3279844522,
0.2431274951,
-0.2901174426,
-0.0558713637,
0.2372847497,
-0.3406096101,
0.5479468703,
0.1450075209,
-0.2062623352,
-0.2959771156,
-0.1340129226,
-0.0942152813,
-0.2200795412,
-0.2425168753,
0.0989219174,
0.1037222669,
-0.1725025177,
0.0372333378,
-0.0137784779,
-0.4823435247,
0.0696524605,
0.0511413068,
0.6196217537,
0.3521491885,
0.0815302208,
-0.3057150841,
0.0834969804,
0.4136028886,
-0.4095362425,
-0.2688110173,
0.1824417114,
0.043478217,
0.2113898695,
-0.2620835006,
-0.3617443442,
0.0265492983,
-0.2976146638,
0.6853888035,
0.0961093009,
-0.0340798497,
-0.16203475,
-0.0633250847,
0.1737491339,
-0.323364377,
0.3805909753,
-0.2930783629,
-0.6595548987,
0.5047426224,
-0.1025177613,
-0.2188171893,
-0.0036960412,
0.0586480908,
0.3344173729,
-0.0894474387,
-0.6739205718,
-0.4296788275,
0.0067402138,
0.26610291,
-0.0254286919,
0.1197581515,
0.5252694488,
-0.0839202628,
0.013743327,
-0.0674875453,
-0.2023646384,
0.5829994678,
-0.0621240065,
0.1375799775,
-0.0825308263,
0.3729040623,
0.1241422892,
0.9117967486,
0.4719625711,
0.3536292911,
0.2457000762,
0.092000559,
0.3813194036,
-0.147935763,
-0.112925224,
0.3361160457,
0.2327179313,
-0.1589663476,
0.2437697202,
0.1992548108,
0.1921728551,
-0.0608862117,
-0.0730624869,
-0.3863713741,
-0.3076613843,
-0.0325331017,
-0.0471555702,
0.2321032584,
-0.006995779,
0.0424785092,
-0.2280808091,
0.0112634236,
0.4220141768,
0.4312684238,
0.2322889268,
0.2378757894,
-0.1441175789,
0.0589373931,
-0.5730266571,
0.2734693289,
-0.5298242569,
0.0832476914,
-0.2206546813,
-0.3305586576,
0.151006043,
0.0413571224,
0.5898936391,
0.177894935,
-0.1399521381,
-0.0725889653,
-0.2002184242,
-0.4257734418,
-0.1523621678,
-0.1288875043,
-0.1171435714,
0.368168354,
0.3341630399,
-0.3990162313,
-0.1482418776,
-0.1328411996,
-0.0242101438,
-0.2809098363,
-0.3642935157,
-0.299569428,
0.055841554,
-0.2109502703,
0.0794523805,
-0.2678174078,
-0.0450141914,
0.0515149087,
0.0376875624,
-0.1677216887,
-0.1312993318,
0.0200151782,
0.074441433,
0.3540907502,
-0.3674109876,
0.1572969556,
0.470513016,
-0.0993364826,
0.0339576416,
0.3758528829,
0.0386760943,
0.0075478577,
0.323161602,
-0.1632549465,
0.2435812801,
0.5914888978,
-0.3155204654,
0.0485773496,
0.0017070374,
0.4769369364,
-0.1545542032,
-0.167685017,
0.3743359447,
-0.1851759255,
0.3994727135,
0.2172225565,
0.1465721577,
0.230744943,
-0.1425453871,
0.2136994302,
0.0921446458,
-0.1486644894,
0.4187479317,
-0.0191746335,
1.0156927109,
0.2073532939,
0.282700032,
0.1329190731,
0.177216351,
0.4591485262,
0.1079503968,
0.0556261614,
-0.2091246843,
-0.2929943502,
0.0010578765,
-0.1498104185,
-0.0807726309,
-0.2313540131,
-0.2762809098,
0.4570981264,
-0.3854296207,
-0.1603197902,
-0.1962326765,
-0.2233335078,
-0.3173438311,
-0.1051385477,
0.0847922936,
-0.0389763154,
0.1487232149,
0.5471584797,
-0.2226289064,
-0.1768107116,
-0.3493129313,
-0.3877287805,
-0.1265835464,
-0.0337560251,
-0.2098590732,
0.0311236419,
0.3574777842,
-0.1190104336,
-0.2099217027,
0.2792098224,
0.3486991823,
0.1478071362,
-0.3286239803,
-0.0536579229,
0.1387420446,
0.0693157613,
0.1134881601,
-0.0162833128,
0.2863363028,
-0.0592634566,
-0.0206089914,
0.0139436256,
-0.2617380917,
0.0625095367,
0.0623125136,
-0.1763907522,
-0.2089602351,
-0.046376761,
-0.0278088227,
0.0901818275,
-0.0493520238,
-0.2061767876,
0.0301746167,
0.3500424027,
-0.2381432205,
0.0757837966,
0.1948085874,
-0.2536815107,
-0.1046508998,
0.5353069901,
-0.2839658856,
-0.0404861793,
0.525798738,
0.0521447808,
-0.0739264339,
-0.0879663676,
0.123145178,
0.3842420578,
-0.8295776248,
0.0623211153,
-0.0147830602,
0.0206480641,
0.0930652022,
0.0981902033,
0.4433167577,
-0.1498864591,
-0.1371233016,
-0.2625293732,
-0.2995174825,
0.2950274646,
-0.3477450013,
0.1463381201,
-0.439057529,
0.0171230175,
-0.1422358751,
0.1940518469,
-0.2237335294,
0.1365992576,
-0.2280649096,
-0.1690291911,
-0.1042845249,
-0.0442445315,
0.3885757625,
-0.0331424661,
-0.0840899348,
0.0814567208,
-0.3113949001,
-0.0289357435,
-0.2361382544,
0.176245451,
-0.1520952135,
0.1542672962,
-0.0213325322,
0.2418330461,
-0.0256441664,
-0.1351262033,
0.0199909303,
0.1746336818,
0.0819576085,
0.18294999,
0.1014146283,
0.109610185,
-0.1310425997,
0.2293001264,
0.0827873945,
-0.2001679689,
-0.0907211229,
0.0441641025,
-0.4078600109,
-0.051729016,
0.1881526858,
0.1526674628,
-0.2726953924,
0.1853770465,
0.0912857577,
-0.0026346906,
-0.3078104854,
0.0038791832,
0.4702508152,
0.1285598427,
-0.2048876286,
0.0074319555,
-0.0171012692,
0.052266404,
-0.1066368818,
0.256721288,
0.080027096,
0.4084863961,
-0.0540617928,
0.2842747867,
0.1380125284,
-0.0800258592,
0.0952326879,
0.2558291256,
-0.073755756,
-0.1462480128,
0.3656685948,
-0.0703355372,
0.0768481717,
0.3500857353,
0.4774136245,
0.110192351,
0.1348931491,
-0.0343454853,
-0.0622372627,
0.1586262584,
-0.0870699584,
0.2827892601,
-0.1443687528,
-0.2000664622,
-0.1374968141,
0.094621852,
0.0407015271,
-0.1743672937,
-0.4197376668,
0.771767199,
-0.2196433246,
-0.242641747,
-0.1869887561,
0.218761459,
-0.1312939227,
-0.1028854102,
-0.1330425441,
-0.1627591252,
-0.188194707,
-0.1369979978,
0.2413046956,
0.0748139918,
0.0135708945,
0.1831948608,
0.0779205412,
-0.2401614636,
-0.409724623,
0.1471296102,
0.2348364443,
-0.1723956168,
0.0443611071,
0.2696974277,
-0.2988670766,
0.182658717,
0.604993999,
0.3143847287,
0.0188652333,
-0.1037894189,
0.1164325625,
0.2726610303,
0.0104301181,
-0.1231353357,
0.1442329288,
0.2494452894,
-0.4571604729,
0.0450881608,
0.0002660026,
-0.1097637042,
0.4263655543,
-0.2372505963,
0.3066157699,
-0.1923082024,
0.4076458216,
-0.1710710078,
0.10759563,
0.0466247834,
-0.1395911574,
-0.7387019992,
0.2041077465,
-0.1014891863,
0.0151313255,
0.0688965544,
-0.2466748208,
0.0107893245,
-0.1486223042,
0.1965497136,
0.5074283481,
0.063035138,
-0.2921724021,
-0.1924870908,
-0.494107455,
0.3129242957,
0.1277842969,
0.2551855445,
-0.0394151099,
0.3570332229,
-0.0903415084,
0.0310945846,
0.2803322971,
-0.0243285857,
-0.1102451831,
0.033442121,
-0.4978340864,
0.1731981635,
-0.0518374369,
-0.0960943177,
0.0645288154,
0.2707465291,
-0.0446341671,
-0.29148072,
-0.0436215401,
0.263794899,
-0.2875217795,
-0.1453558356,
0.1927304417,
0.4304180145,
0.1727125645,
0.1035243198,
-0.0241545569,
-0.144763127,
-0.1757726073,
-0.1006725207,
-0.0385694504,
-0.1139790565,
-0.2793590128,
0.4239610136,
-0.3997518718,
-0.4653258324,
-0.3727270663,
0.3133133352,
0.0486241132,
-0.1338502467,
-0.2125531882,
0.3537250459,
-0.1586045921,
0.0163045544,
0.2923789322,
0.274410069,
-0.0121015897,
0.3496613503,
-0.2905235589,
-0.1873505265,
0.7223866582,
-0.5237226486,
0.3236786425,
-0.266492337,
0.4198945761,
0.3544080853,
-0.3350405991,
-0.3600741029,
-0.0202660672,
0.127798453,
0.08860939,
-0.15515697,
0.0890783221,
-0.3894801736,
-0.0616634153,
0.0164005198,
0.239749074,
0.1686126292,
-0.0078574549,
-0.0522723421,
-0.1597507447
]
|
https://github.com/huggingface/datasets/issues/1942 | [experiment] missing default_experiment-1-0.arrow | are you sure you need an external lock file? if it's a single purpose locking in the same scope you can lock the caller `__file__` instead, e.g. here is how one can `flock` the script file itself to ensure atomic printing:
```
import fcntl
def printflock(*msgs):
""" print in multiprocess env so that the outputs from different processes don't get interleaved """
with open(__file__, "r") as fh:
fcntl.flock(fh, fcntl.LOCK_EX)
try:
print(*msgs)
finally:
fcntl.flock(fh, fcntl.LOCK_UN)
```
| the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you. | 75 | [experiment] missing default_experiment-1-0.arrow
the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you.
are you sure you need an external lock file? if it's a single purpose locking in the same scope you can lock the caller `__file__` instead, e.g. here is how one can `flock` the script file itself to ensure atomic printing:
```
import fcntl
def printflock(*msgs):
""" print in multiprocess env so that the outputs from different processes don't get interleaved """
with open(__file__, "r") as fh:
fcntl.flock(fh, fcntl.LOCK_EX)
try:
print(*msgs)
finally:
fcntl.flock(fh, fcntl.LOCK_UN)
```
| [
-0.0958080515,
-0.0942350626,
0.1255572438,
0.0927404538,
-0.0868550539,
-0.0296323933,
0.2317222357,
0.2375227213,
0.2348065674,
0.1782364547,
0.0034629612,
0.1474096775,
-0.2378917038,
-0.0178875886,
-0.0204138737,
0.1772463918,
0.0075173462,
-0.0802014247,
-0.3421195745,
-0.1448379904,
-0.2741203308,
0.5315679908,
-0.1308237463,
-0.0719343871,
-0.4290017486,
0.2123155296,
-0.2263915092,
0.1211147755,
-0.0081583997,
-0.3395676613,
0.1049850211,
0.3507534266,
0.1030158326,
0.4625882804,
-0.0001223227,
-0.2479463518,
0.1706752032,
-0.1718241572,
-0.101175487,
-0.0645022467,
0.1405714899,
-0.310826391,
0.4765367806,
-0.2978206575,
0.1028481647,
0.0841090977,
0.1408373564,
-0.3395662606,
0.4358517528,
-0.0378724299,
0.0893843621,
0.2628899813,
0.1200162768,
-0.177528441,
-0.0331903324,
-0.1780334413,
0.0121775866,
0.270599097,
-0.1119369715,
0.0844490603,
-0.2585884333,
0.2509045899,
0.2237696201,
-0.2024631053,
0.1423095763,
0.0610954128,
-0.1400079727,
-0.2547034919,
-0.1634259373,
0.2697405517,
0.1364804655,
-0.3204967082,
-0.3008386493,
0.0492604338,
-0.117218487,
-0.3431064487,
0.0262464974,
-0.0340271071,
-0.0005132668,
0.0618581697,
0.1232935712,
-0.1993052959,
-0.0104408721,
0.0003192303,
-0.0139873456,
0.0958360583,
-0.1606515199,
0.0895802006,
-0.1322296709,
0.2888589799,
-0.3445693552,
0.387701422,
0.0099182017,
0.1042268649,
-0.2629314363,
0.1574040204,
0.2871651947,
-0.1044999212,
0.1025783122,
0.3618664742,
-0.0343257748,
0.0118683605,
0.535415709,
0.1580042392,
-0.0143521866,
0.3216005564,
0.2761748433,
0.01939773,
0.2049499303,
0.400488168,
-0.2367349714,
-0.2947703004,
0.2996624708,
-0.2098306865,
0.3451395929,
0.1116674021,
0.1468065232,
-0.4740607738,
0.3149006367,
0.3492856622,
-0.0679859594,
-0.0676053762,
-0.027408218,
0.3686688542,
0.2322142273,
0.0972461179,
0.3592592478,
0.221901685,
-0.2242744714,
-0.0752862766,
-0.3204059601,
-0.1706392765,
-0.0248743016,
0.407238543,
-0.077752769,
-0.0594258159,
0.529573679,
-0.0586508997,
0.5135623813,
-0.088576071,
0.0644365922,
0.1758890301,
0.0725542903,
0.2391678393,
0.0254030451,
0.235724166,
0.3454735279,
0.2439837307,
-0.2318498343,
-0.3414820135,
0.0639522076,
-0.4619215131,
0.105822742,
-0.0182387922,
-0.3980557024,
0.1951526403,
0.6058905721,
0.1725613028,
-0.1805833429,
-0.2601391971,
0.311419338,
0.0852637589,
-0.0760382339,
-0.0874108821,
0.4565060735,
0.7431333065,
-0.3583600819,
-0.3069775403,
0.1075282395,
-0.1093930602,
0.0635072514,
0.1491248459,
0.0973034874,
0.0965095609,
-0.2766649723,
-0.1178214625,
0.357141763,
-0.4726915061,
-0.472574532,
0.0852728337,
-0.4719014764,
-0.0274128132,
0.206682384,
0.007604077,
0.2238253206,
0.2170167714,
0.1575412601,
-0.1981642544,
0.2113953978,
-0.2767271399,
-0.3929211199,
-0.1785396487,
-0.1324971765,
-0.0649427027,
0.2047933787,
0.0115920631,
0.0840650499,
0.0313536562,
0.2286922783,
0.0897835493,
0.1888239235,
0.2832201719,
0.3775961697,
0.1742913127,
0.2464332432,
0.1135072112,
-0.2764573991,
0.2135855705,
-0.4569099545,
0.1178639755,
0.0813399404,
-0.0489260964,
-0.0218003448,
-0.1058619693,
-0.0418674797,
-0.414740026,
0.0085284738,
-0.0434775241,
0.3030488491,
0.2495978624,
-0.179623425,
0.2385919094,
-0.0130313011,
0.2911893725,
-0.4203337729,
-0.1497297585,
-0.0477625057,
-0.388225466,
0.0575976931,
0.1718962342,
0.0015684104,
0.0748588294,
-0.1377815604,
0.4197940826,
-0.0571035147,
0.2876298726,
0.2521413565,
0.1678893566,
-0.197128281,
-0.2420714051,
-0.0125149637,
0.065709278,
-0.0356096812,
0.0254986659,
-0.1123304218,
0.490195632,
0.0970407724,
0.0857904553,
-0.3372977078,
0.2576317191,
-0.1290614456,
-0.0623217411,
-0.5252737403,
-0.1358708143,
0.3787255585,
-0.2251190245,
0.2366427332,
-0.1315061599,
0.0301114917,
0.0798023939,
0.1785760522,
0.2459707856,
0.1715172529,
-0.0005888969,
-0.1394902468,
-0.0125994477,
0.0347565301,
-0.0848472118,
0.2582924962,
0.2357060611,
0.1050706655,
0.0881277919,
0.1087511107,
-0.1732835919,
0.1077508703,
-0.0638256744,
-0.1016962379,
0.1692504287,
0.1857384443,
0.1188375577,
-0.2927374244,
-0.1776987016,
-0.3255642653,
-0.0320764035,
-0.2366853654,
-0.0204164479,
0.0345231853,
0.3458486199,
0.0091696568,
0.0865001902,
-0.2900827825,
-0.4045255482,
0.2174940854,
-0.1798907071,
-0.0688814223,
0.1823812872,
-0.4417122006,
0.4122615457,
0.1074885353,
-0.241872564,
-0.32942307,
-0.1597178876,
-0.0786581188,
-0.2074676454,
-0.2837310135,
0.1251960397,
0.2220121026,
-0.0486113094,
0.0800969526,
-0.1392988712,
-0.3460685611,
0.0014420042,
-0.0510541126,
0.6285652518,
0.291164428,
-0.0305411555,
-0.1663736254,
0.0547244288,
0.4496113658,
-0.5593895912,
-0.1918305308,
0.1030514836,
-0.0518235601,
0.1852311343,
-0.2551889122,
-0.2687278092,
0.0448058881,
-0.414847821,
0.6636123657,
0.0234175865,
-0.0101745175,
-0.223594144,
-0.1076808423,
0.178101927,
-0.2553530335,
0.4349594116,
-0.1497937292,
-0.5847467184,
0.4244234264,
-0.1281864047,
-0.2230436802,
-0.1408716142,
0.0822280422,
0.1759923398,
-0.1449052095,
-0.5932176113,
-0.5006263256,
-0.1014444008,
0.2448943853,
-0.0263821315,
0.1439138353,
0.4210921228,
-0.1415074915,
-0.0179312415,
-0.012903491,
-0.1239338294,
0.5327204466,
-0.1963026673,
0.0532473139,
-0.0795467645,
0.4616315961,
0.0842702165,
0.8287322521,
0.5620364547,
0.3317268789,
0.1832953691,
0.021969799,
0.4228359759,
-0.1368256658,
-0.1904709488,
0.3991368413,
0.3047936261,
-0.2714654803,
0.2112304121,
0.0435510203,
0.2230719179,
-0.1163756922,
0.0106749544,
-0.4465950131,
-0.4564573467,
-0.0719128475,
0.0920202956,
0.2174005061,
-0.0941522047,
0.0339870378,
-0.0975618139,
0.0202384181,
0.5146847367,
0.3764463067,
0.1631000787,
0.127796635,
-0.1811332703,
-0.0057473634,
-0.6595858335,
0.3577419221,
-0.4646303654,
0.1572023928,
-0.1583257616,
-0.3413120806,
0.1660343409,
-0.0564943887,
0.6355431676,
0.1735780984,
-0.1956251711,
-0.0046773679,
-0.254747957,
-0.4369533658,
-0.102799505,
-0.2421706617,
-0.150762096,
0.3620061278,
0.0918475315,
-0.4735631049,
-0.132445395,
-0.1301804781,
-0.1474504471,
-0.3104039133,
-0.3958632946,
-0.2934835255,
0.0456177145,
-0.3154398203,
0.0738437921,
-0.3079229295,
0.0215212647,
0.01810937,
0.0927207023,
0.0271872375,
-0.1077788249,
0.0773515254,
0.1022578105,
0.2887766361,
-0.2136120498,
0.0983375013,
0.4372387826,
-0.1915365905,
-0.0192966126,
0.3132376969,
0.1018751934,
-0.0999715403,
0.1759303659,
-0.0794544667,
0.279962033,
0.603248477,
-0.1729379743,
0.0549091995,
-0.0586395487,
0.4818812013,
-0.1178968102,
-0.2855543792,
0.2373218387,
-0.1547118127,
0.3927036226,
0.2192186117,
0.0485758483,
0.3099293411,
-0.1057201028,
0.3555971682,
-0.0205978844,
-0.1411520243,
0.4236705303,
0.1191987321,
0.9506545663,
0.097658135,
0.1860413849,
0.2422057539,
-0.0376852453,
0.449213624,
0.0296555366,
0.0142840473,
-0.3872033656,
-0.2756678164,
0.0917525068,
-0.1032315195,
-0.0816481709,
-0.3444143534,
-0.2914730012,
0.3155267835,
-0.3911910057,
-0.3037450314,
-0.3794370294,
-0.1960083991,
-0.4446932971,
-0.2635650635,
-0.0477045737,
-0.0090243462,
0.1433114707,
0.5026946664,
-0.3078815341,
-0.1107046455,
-0.288667649,
-0.3712599576,
-0.2273341417,
-0.0222585797,
-0.2027353793,
-0.0640976578,
0.2401202768,
0.091819182,
-0.3199107945,
0.3298313022,
0.3815290332,
0.1273177713,
-0.2358823866,
-0.1780479401,
0.2861911654,
0.0382467136,
0.1124104112,
0.001428836,
0.2514291704,
0.0359149612,
-0.0307592601,
0.1709093302,
-0.1664932221,
0.0872223079,
0.1005963087,
-0.1597257853,
-0.1423914284,
-0.1958402246,
-0.0657534897,
0.1554857939,
-0.0663395673,
-0.210457027,
0.0482373312,
0.2457900792,
-0.314684093,
0.1481902003,
0.0379268005,
-0.2771350741,
-0.0750765055,
0.5247075558,
-0.264031738,
-0.0248467475,
0.3970453441,
0.2828392684,
-0.0860161707,
-0.1441111118,
0.1293325424,
0.298658818,
-0.7690412402,
0.2018031627,
0.1477664113,
-0.0192423407,
0.0434803739,
0.2532588542,
0.3413796127,
-0.1568588167,
-0.095513843,
-0.2206640989,
-0.3461401463,
0.3158051372,
-0.2180737108,
0.2099336982,
-0.2942487299,
0.1017864496,
-0.1563839018,
0.2841729522,
-0.2742086649,
0.0098687736,
-0.2385792434,
-0.1825030595,
-0.0759446174,
-0.0353174992,
0.3743842244,
0.1295119375,
-0.0323033407,
0.222045362,
-0.2932639122,
-0.0894105285,
-0.2077835053,
0.1688524038,
-0.1008316651,
0.227456972,
-0.0931679606,
0.1616181135,
-0.1409088522,
-0.1505561769,
0.1215030849,
0.3491714597,
0.0348306559,
0.157963559,
0.2235541195,
0.052499067,
-0.1588916481,
0.1782958955,
0.0967275798,
-0.164704591,
-0.0673634782,
0.023814993,
-0.4683922827,
-0.0754993632,
0.1976671815,
0.1554302722,
-0.1301897019,
0.1547017097,
0.0438504219,
0.0733111352,
-0.3498416543,
0.0681979284,
0.5667786598,
0.2293316126,
-0.194715187,
-0.0845657885,
-0.0761870146,
0.0748456717,
-0.079774417,
0.4089384377,
0.0196244866,
0.2220223099,
0.0884397477,
0.3918869495,
0.0069049522,
-0.1549844593,
0.0887219459,
0.1567454189,
-0.1267596036,
-0.1499550492,
0.2738580108,
-0.0977541581,
0.1879411787,
0.3699200749,
0.5017706752,
0.1857876182,
0.1736929715,
-0.1599157602,
-0.0085363341,
-0.0041765901,
-0.1832285076,
0.3578149676,
-0.0631647557,
-0.1351079792,
-0.061901696,
0.0247355085,
0.0999882147,
-0.1619317532,
-0.4995583296,
0.7212762833,
-0.1596979648,
-0.0727414936,
-0.1729461551,
0.4202396274,
-0.1257976443,
-0.1201534569,
-0.0807233602,
-0.1388193369,
-0.0810432211,
-0.1081054956,
0.3586573899,
0.1451634318,
0.0638324022,
0.2073589712,
0.1183852777,
-0.2788422704,
-0.4896350205,
0.2174435407,
0.2856248915,
-0.2475684136,
0.0128943389,
0.2346802205,
-0.2322444171,
0.1432936043,
0.6515596509,
0.2464318424,
0.0117480354,
-0.0120476838,
0.2025605291,
0.2649377882,
0.0501917154,
-0.1223697513,
0.164022848,
0.2685832977,
-0.457116127,
0.2159977853,
0.043723546,
-0.1576762795,
0.2495297492,
-0.2333385348,
0.2681140602,
-0.0029877445,
0.5281679034,
-0.0881278217,
0.2892680466,
-0.0906342641,
-0.1573892534,
-0.6749103665,
0.1098422855,
-0.1533481777,
-0.1440504789,
0.039729178,
-0.3130023181,
0.0267413855,
-0.0766397715,
0.0271467417,
0.5202211142,
0.1198401079,
-0.2506418824,
-0.2004323006,
-0.4098306596,
0.3709433675,
0.1497469693,
0.1613406837,
-0.0727337003,
0.4148483574,
-0.0166737363,
0.078647159,
0.2746063769,
-0.0772274211,
-0.2901545763,
0.2360419184,
-0.42977494,
0.2515310347,
-0.2711085081,
-0.0724578127,
0.073643066,
0.3046460748,
-0.0841523856,
-0.2813908458,
-0.0065455092,
0.2192519307,
-0.2696210742,
-0.1493306607,
0.0387560129,
0.3670335114,
0.1418908983,
0.0027358227,
-0.124559246,
-0.1818846166,
-0.1920180917,
-0.0750166401,
-0.1639818251,
-0.1211035475,
-0.2894135118,
0.4088885188,
-0.3357613981,
-0.5149748921,
-0.3786816895,
0.3133417368,
0.1088765636,
-0.1906794608,
-0.1274160892,
0.3311881125,
-0.1567311138,
0.0528099015,
0.2602566779,
0.4714580774,
0.0496160872,
0.4661210179,
-0.2932114899,
-0.1922186166,
0.6927307248,
-0.4649078548,
0.2622733712,
-0.1952013224,
0.4434484243,
0.1342340261,
-0.3789175749,
-0.3714775443,
0.0214508288,
0.0577610619,
0.1420639902,
-0.0396044739,
0.1356397718,
-0.3098531961,
-0.1109305918,
-0.0667540729,
0.182085827,
0.1642800719,
0.1262944639,
-0.1123882011,
-0.0309418291
]
|
https://github.com/huggingface/datasets/issues/1942 | [experiment] missing default_experiment-1-0.arrow | OK, this issue is not about caching but some internal conflict/race condition it seems, I have just run into it on my normal env:
```
Traceback (most recent call last):
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/metric.py", line 356, in _finalize
self.data = Dataset(**reader.read_files([{"filename": f} for f in file_paths]))
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/arrow_reader.py", line 236, in read_files
pa_table = self._read_files(files, in_memory=in_memory)
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/arrow_reader.py", line 171, in _read_files
pa_table: pa.Table = self._get_dataset_from_filename(f_dict, in_memory=in_memory)
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/arrow_reader.py", line 302, in _get_dataset_from_filename
pa_table = ArrowReader.read_table(filename, in_memory=in_memory)
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/arrow_reader.py", line 322, in read_table
stream = stream_from(filename)
File "pyarrow/io.pxi", line 782, in pyarrow.lib.memory_map
File "pyarrow/io.pxi", line 743, in pyarrow.lib.MemoryMappedFile._open
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 97, in pyarrow.lib.check_status
FileNotFoundError: [Errno 2] Failed to open local file '/home/stas/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow'. Detail: [errno 2] No such file or directory
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "examples/seq2seq/run_seq2seq.py", line 655, in <module>
main()
File "examples/seq2seq/run_seq2seq.py", line 619, in main
test_results = trainer.predict(
File "/mnt/nvme1/code/huggingface/transformers-master/src/transformers/trainer_seq2seq.py", line 121, in predict
return super().predict(test_dataset, ignore_keys=ignore_keys, metric_key_prefix=metric_key_prefix)
File "/mnt/nvme1/code/huggingface/transformers-master/src/transformers/trainer.py", line 1706, in predict
output = self.prediction_loop(
File "/mnt/nvme1/code/huggingface/transformers-master/src/transformers/trainer.py", line 1813, in prediction_loop
metrics = self.compute_metrics(EvalPrediction(predictions=preds, label_ids=label_ids))
File "examples/seq2seq/run_seq2seq.py", line 556, in compute_metrics
result = metric.compute(predictions=decoded_preds, references=decoded_labels)
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/metric.py", line 388, in compute
self._finalize()
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/metric.py", line 358, in _finalize
raise ValueError(
ValueError: Error in finalize: another metric instance is already using the local cache file. Please specify an experiment_id to avoid colision between distributed metric instances.
```
I'm just running `run_seq2seq.py` under DeepSpeed:
```
export BS=16; rm -r output_dir; PYTHONPATH=src USE_TF=0 CUDA_VISIBLE_DEVICES=0,1 deepspeed --num_gpus=2 examples/seq2seq/run_seq2seq.py --model_name_or_path t5-small --output_dir output_dir --adam_eps 1e-06 --do_eval --do_train --do_predict --evaluation_strategy=steps --label_smoothing 0.1 --learning_rate 3e-5 --logging_first_step --logging_steps 1000 --max_source_length 128 --max_target_length 128 --num_train_epochs 1 --overwrite_output_dir --per_device_eval_batch_size $BS --per_device_train_batch_size $BS --predict_with_generate --eval_steps 25000 --sortish_sampler --task translation_en_to_ro --val_max_target_length 128 --warmup_steps 500 --max_train_samples 100 --max_val_samples 100 --max_test_samples 100 --dataset_name wmt16 --dataset_config ro-en --source_prefix "translate English to Romanian: " --deepspeed examples/tests/deepspeed/ds_config.json
```
It finished the evaluation OK and crashed on the prediction part of the Trainer. But the eval / predict parts no longer run under Deepspeed, it's just plain ddp.
Is this some kind of race condition? It happens intermittently - there is nothing else running at the same time.
But if 2 independent instances of the same script were to run at the same time it's clear to see that this problem would happen. Perhaps it'd help to create a unique hash which is shared between all processes in the group and use that as the default experiment id?
| the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you. | 409 | [experiment] missing default_experiment-1-0.arrow
the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you.
OK, this issue is not about caching but some internal conflict/race condition it seems, I have just run into it on my normal env:
```
Traceback (most recent call last):
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/metric.py", line 356, in _finalize
self.data = Dataset(**reader.read_files([{"filename": f} for f in file_paths]))
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/arrow_reader.py", line 236, in read_files
pa_table = self._read_files(files, in_memory=in_memory)
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/arrow_reader.py", line 171, in _read_files
pa_table: pa.Table = self._get_dataset_from_filename(f_dict, in_memory=in_memory)
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/arrow_reader.py", line 302, in _get_dataset_from_filename
pa_table = ArrowReader.read_table(filename, in_memory=in_memory)
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/arrow_reader.py", line 322, in read_table
stream = stream_from(filename)
File "pyarrow/io.pxi", line 782, in pyarrow.lib.memory_map
File "pyarrow/io.pxi", line 743, in pyarrow.lib.MemoryMappedFile._open
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 97, in pyarrow.lib.check_status
FileNotFoundError: [Errno 2] Failed to open local file '/home/stas/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow'. Detail: [errno 2] No such file or directory
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "examples/seq2seq/run_seq2seq.py", line 655, in <module>
main()
File "examples/seq2seq/run_seq2seq.py", line 619, in main
test_results = trainer.predict(
File "/mnt/nvme1/code/huggingface/transformers-master/src/transformers/trainer_seq2seq.py", line 121, in predict
return super().predict(test_dataset, ignore_keys=ignore_keys, metric_key_prefix=metric_key_prefix)
File "/mnt/nvme1/code/huggingface/transformers-master/src/transformers/trainer.py", line 1706, in predict
output = self.prediction_loop(
File "/mnt/nvme1/code/huggingface/transformers-master/src/transformers/trainer.py", line 1813, in prediction_loop
metrics = self.compute_metrics(EvalPrediction(predictions=preds, label_ids=label_ids))
File "examples/seq2seq/run_seq2seq.py", line 556, in compute_metrics
result = metric.compute(predictions=decoded_preds, references=decoded_labels)
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/metric.py", line 388, in compute
self._finalize()
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/metric.py", line 358, in _finalize
raise ValueError(
ValueError: Error in finalize: another metric instance is already using the local cache file. Please specify an experiment_id to avoid colision between distributed metric instances.
```
I'm just running `run_seq2seq.py` under DeepSpeed:
```
export BS=16; rm -r output_dir; PYTHONPATH=src USE_TF=0 CUDA_VISIBLE_DEVICES=0,1 deepspeed --num_gpus=2 examples/seq2seq/run_seq2seq.py --model_name_or_path t5-small --output_dir output_dir --adam_eps 1e-06 --do_eval --do_train --do_predict --evaluation_strategy=steps --label_smoothing 0.1 --learning_rate 3e-5 --logging_first_step --logging_steps 1000 --max_source_length 128 --max_target_length 128 --num_train_epochs 1 --overwrite_output_dir --per_device_eval_batch_size $BS --per_device_train_batch_size $BS --predict_with_generate --eval_steps 25000 --sortish_sampler --task translation_en_to_ro --val_max_target_length 128 --warmup_steps 500 --max_train_samples 100 --max_val_samples 100 --max_test_samples 100 --dataset_name wmt16 --dataset_config ro-en --source_prefix "translate English to Romanian: " --deepspeed examples/tests/deepspeed/ds_config.json
```
It finished the evaluation OK and crashed on the prediction part of the Trainer. But the eval / predict parts no longer run under Deepspeed, it's just plain ddp.
Is this some kind of race condition? It happens intermittently - there is nothing else running at the same time.
But if 2 independent instances of the same script were to run at the same time it's clear to see that this problem would happen. Perhaps it'd help to create a unique hash which is shared between all processes in the group and use that as the default experiment id?
| [
-0.0576909408,
-0.0025042647,
0.1063461304,
0.3295958042,
-0.0730611458,
0.0291780587,
0.1474633217,
0.2418281585,
0.3056666851,
0.1599035561,
0.2047880888,
0.07196071,
-0.3148393929,
-0.1378425509,
-0.0102977818,
0.2024057209,
0.0270130634,
0.0037931344,
-0.2088185698,
-0.1798237711,
-0.323795557,
0.5144023895,
-0.0927539244,
-0.0305796117,
-0.3576315045,
0.3367960453,
-0.1782272011,
0.302488476,
0.0206065904,
-0.6091863513,
0.4519521892,
0.1594463736,
0.1245750263,
0.4872968197,
-0.0001303917,
-0.1621837318,
0.2800585032,
-0.1859594136,
-0.1424783468,
-0.2011278272,
-0.0963114873,
-0.2803774476,
0.4510984123,
-0.2343736589,
-0.0237274896,
-0.0773368031,
0.1758155972,
-0.591581583,
0.3542674184,
0.113642998,
-0.0048830649,
0.3083098829,
-0.1144850478,
-0.2425566018,
0.1217076331,
-0.0425466821,
0.0690077096,
0.2845218182,
-0.086033456,
-0.1388477683,
-0.2688546479,
0.2733640373,
0.1988229454,
-0.054087799,
0.4352083206,
0.0169219393,
0.0313850157,
-0.0497768261,
-0.1019930765,
0.3329291642,
0.1740123034,
-0.193828389,
-0.208735466,
0.0363136269,
-0.1447733492,
-0.4275948405,
0.2937576175,
-0.0938257575,
0.0557494573,
0.1103092358,
0.0745092854,
-0.0691117719,
0.0193977468,
0.1733286679,
-0.1224160865,
0.1237847358,
-0.0248198397,
0.0248816926,
-0.0011232669,
0.2460563034,
-0.1643097848,
0.1366793662,
-0.089115873,
0.2079615444,
-0.3737041652,
0.1329749823,
0.1770111024,
-0.0221960749,
-0.0012672486,
0.2791175842,
-0.0404294021,
0.0242971573,
0.3617475927,
0.1396158785,
-0.01197745,
0.4315190315,
0.2799912095,
0.1480381191,
0.2010221481,
0.2454795092,
-0.0930143446,
-0.1489389688,
0.1177478284,
-0.2112674564,
0.3843247294,
0.1562825739,
-0.0006583772,
-0.4661146402,
0.068159245,
0.3652066588,
-0.2267410457,
-0.1440908313,
0.2043337524,
0.3926242888,
0.196039781,
-0.036498636,
0.1631371528,
0.2825306952,
-0.2508918047,
-0.0268497691,
-0.4424969256,
-0.2588557899,
-0.1373983175,
0.2259522229,
0.1951781362,
-0.0326907858,
0.5749141574,
-0.2132206112,
0.3801061809,
-0.0748555586,
0.2046854794,
0.100337863,
0.0419852845,
0.2588789761,
-0.077568084,
0.2467136681,
0.4501028955,
0.0853140503,
-0.2225541621,
-0.1783109456,
-0.1147633418,
-0.5404672623,
0.1880502701,
-0.0822354332,
-0.4932709336,
0.2703610957,
0.4431523979,
0.158653006,
0.0580167696,
-0.340962708,
0.2449740022,
-0.0816508755,
-0.2438094169,
-0.0823498741,
0.4338891506,
0.7405844331,
-0.301900059,
-0.3919821084,
0.0709852278,
0.1103326678,
-0.0848061144,
0.2470315397,
-0.167689845,
-0.05899271,
-0.3711158633,
0.0297603533,
0.3290180266,
-0.4905430973,
-0.6476852298,
-0.1112069711,
-0.3637507558,
-0.0950269252,
0.2161112875,
0.140177682,
0.0239368025,
-0.056367822,
0.1230907366,
-0.174112469,
0.2157108039,
-0.3362264931,
-0.2547810376,
-0.3030844629,
0.0921702385,
0.0116520319,
0.3164172769,
-0.069231905,
-0.0339648314,
-0.1159877479,
0.1047610566,
0.1246333867,
0.1933833808,
0.3314184844,
0.2837238312,
-0.0269378219,
0.3410243094,
0.2112777531,
-0.3488749564,
0.3641499877,
-0.5019800067,
0.0062015881,
0.100661613,
0.0107667055,
-0.0791028664,
-0.2361927629,
-0.1411094368,
-0.4627401531,
-0.0980774835,
-0.1100679412,
0.0589428432,
0.1902160197,
-0.1977372766,
0.186808899,
-0.0470207594,
0.2514113784,
-0.4648359418,
0.0201667268,
-0.0818687528,
-0.2810692191,
-0.0736851022,
0.1205330342,
0.0165864546,
0.0521006919,
-0.2455529422,
0.351698935,
-0.0886231065,
0.2942872345,
0.2905912995,
0.2052252591,
-0.0483785793,
-0.4059664905,
-0.0110338815,
0.1969527155,
-0.1073716283,
0.0252720248,
-0.3456171453,
0.4294165671,
0.0680322424,
0.1740291715,
-0.3788431585,
0.1567653269,
-0.1176290065,
-0.1660422683,
-0.4288589358,
-0.1451913118,
0.5433690548,
-0.1508428305,
0.3000461757,
-0.0514612496,
0.051211834,
-0.0064017652,
0.2151769996,
0.2164013237,
0.1246337071,
0.0957659334,
-0.2813656032,
-0.1090493947,
0.0874329135,
0.0135740628,
0.5847156048,
0.197736457,
0.2524624467,
0.1471093148,
0.0353331529,
-0.1976370513,
0.1717870981,
-0.0318983123,
-0.0335674696,
0.2177736759,
0.2405481935,
0.1597720087,
-0.2981689572,
-0.1236057654,
-0.2751498818,
0.0664380267,
-0.3651404679,
0.1122676283,
-0.2560161948,
0.3395060599,
0.1566370875,
0.0014969015,
-0.2696928382,
-0.2396303862,
0.1786822677,
-0.141063571,
0.0234253891,
0.2518823147,
-0.410979718,
0.4310763776,
0.0332371369,
-0.2168575227,
-0.2495375127,
-0.310240984,
-0.1903650016,
-0.2834860981,
-0.111296922,
0.055781696,
0.0091716638,
-0.0543885827,
-0.0524968766,
0.0212188456,
-0.3649505675,
-0.0103321029,
0.1506588757,
0.6111649275,
0.2543147504,
0.0139052551,
-0.3684000373,
0.0578798801,
0.3914336264,
-0.2630778849,
-0.3664732575,
0.0817094818,
0.043145258,
0.2250203192,
-0.2592799067,
-0.3096571863,
0.0280251689,
-0.3285588622,
0.4363334775,
-0.03278777,
0.0186581984,
-0.0697613508,
-0.0786050633,
0.0785870701,
-0.2438894063,
0.1752251834,
-0.3726035357,
-0.5516352654,
0.3954834342,
-0.0112832431,
-0.1737854183,
-0.1263962835,
0.0514002033,
0.3583474457,
0.0741553828,
-0.7520510554,
-0.4106816947,
0.0507171117,
0.2256350517,
-0.1302636117,
-0.0699435398,
0.6061976552,
-0.0339117683,
0.0616026893,
-0.0909519121,
-0.1484256238,
0.6115364432,
-0.1508133858,
0.1900130361,
-0.0960240066,
0.4599851668,
0.207508713,
0.9944368005,
0.5102088451,
0.1887704134,
0.273881048,
0.2238529176,
0.4091356993,
-0.0956352428,
-0.1983337849,
0.2562208176,
0.2452371269,
-0.0865912661,
0.2047559321,
0.0359234102,
0.1220122501,
-0.0613911562,
-0.0637567267,
-0.2538514435,
-0.3101133704,
-0.0132153304,
-0.1616046578,
0.3300978541,
0.0614188015,
0.0212095603,
-0.275708437,
-0.0623923242,
0.3666172326,
0.4859732985,
0.1757145971,
0.2632331848,
-0.2251617014,
0.0044160793,
-0.4434444308,
0.2522541285,
-0.3854750991,
0.2858230472,
-0.2330491841,
-0.1856444776,
0.0981950983,
-0.0833994448,
0.6718569994,
0.1430875957,
-0.0848102346,
-0.0781539232,
-0.0773271322,
-0.3918813467,
-0.1582131684,
0.0495566837,
0.0107547157,
0.2382263243,
0.4341592193,
-0.3735691011,
-0.2426629514,
-0.0838241875,
-0.1122098565,
-0.2599928379,
-0.45254004,
-0.2815353572,
0.144614324,
-0.1928813756,
0.1833468229,
-0.211340785,
-0.0374570265,
0.0612428151,
-0.0035396665,
-0.2129247934,
-0.1019912809,
0.0182372052,
-0.0392022319,
0.3360670507,
-0.4024118781,
0.2283703387,
0.5376233459,
-0.1064091474,
0.0376134105,
0.3673104942,
0.1663351804,
-0.1827933043,
0.2683011889,
-0.0340067036,
0.2161350101,
0.6718816757,
-0.1646303535,
0.1388864815,
0.1991298944,
0.2080637366,
-0.1390984207,
-0.0884976089,
0.3013364971,
-0.1133783758,
0.2461327016,
0.1548595876,
0.2360225022,
0.2951619625,
-0.1596686542,
0.4172233343,
-0.0116959466,
-0.1624190211,
0.2946783006,
0.0393434241,
1.0834723711,
0.292222023,
0.3179516494,
0.135924086,
-0.018277403,
0.3813756704,
0.0950418934,
0.0987549126,
-0.2544327378,
-0.313120991,
-0.065598473,
-0.2473013848,
-0.0368867442,
-0.2755257785,
-0.1136836708,
0.441273123,
-0.2594440877,
-0.0031390528,
-0.1601789743,
-0.2166810185,
-0.2920580506,
-0.1391657442,
0.1713824868,
-0.0610483661,
0.0194268394,
0.5495752692,
-0.1914759278,
-0.1742054075,
-0.428065747,
-0.2743675709,
-0.1341759264,
-0.0437696055,
-0.3516484797,
0.0898429528,
0.2500084639,
-0.3409356773,
-0.1055029705,
0.3168905973,
0.4565446973,
0.1514366567,
-0.3181409538,
0.1140343025,
0.2022185773,
0.1065971628,
0.0955068097,
-0.1106807068,
0.1808834821,
-0.0044128168,
-0.0609437861,
0.0249477029,
-0.2868683934,
0.044536192,
0.0080900583,
-0.1284437925,
-0.0748340115,
0.0710113049,
-0.114834398,
0.086543493,
0.0567271747,
-0.2192519158,
-0.0001807062,
0.3327894509,
-0.1402468979,
-0.0548496358,
0.0633069426,
-0.344289273,
-0.1063786447,
0.7176715136,
-0.0840652287,
-0.1729814112,
0.6388204098,
-0.0108445399,
-0.0114883445,
-0.0330063999,
0.068627201,
0.4259322286,
-0.8050131798,
-0.0211883634,
-0.0666622892,
-0.0668365881,
0.1689559817,
0.0528477319,
0.4529181123,
-0.1656745672,
-0.0827274919,
-0.2919704318,
-0.4071454108,
0.2054396868,
-0.4431144893,
0.2485616654,
-0.3803115785,
-0.0345145836,
-0.3471584618,
0.1759299189,
-0.1712403148,
0.2049067914,
-0.1429181993,
-0.1194588393,
-0.1073106006,
-0.0242531933,
0.2813020349,
-0.1077295393,
-0.1590747237,
0.05602796,
-0.4286453724,
-0.0027017568,
-0.2546127439,
0.2196522951,
-0.0806879848,
0.1286474019,
-0.0928508788,
0.0388119593,
0.0574888252,
-0.1133800223,
-0.0858349875,
0.1680558175,
0.082787469,
0.0316744559,
0.065155603,
0.2205141336,
-0.1086529717,
0.3121110499,
0.0699836016,
-0.2269549966,
-0.0963369086,
0.059795931,
-0.3726486862,
-0.0195324104,
0.0980867967,
0.0736281723,
-0.218891874,
0.1540914327,
0.1128558815,
0.0042943214,
-0.3112638891,
0.0228293091,
0.339736104,
0.0601585954,
-0.1754279137,
-0.0453226902,
0.0236439388,
0.0185236204,
-0.076724194,
0.2651643455,
0.1048921943,
0.4075003564,
0.0353325829,
0.20183599,
0.242906943,
-0.0319001786,
0.1388360709,
0.2965881824,
0.093164593,
-0.2163161486,
0.3850471973,
-0.1120210364,
0.1703716666,
0.335347563,
0.529411912,
0.1533009112,
0.3033120036,
0.0558895208,
-0.0029091712,
0.1036749631,
0.0792402327,
0.2830419242,
-0.2751311064,
-0.2732942104,
-0.2105269432,
0.1802258044,
0.0099640321,
-0.1593453437,
-0.2688837945,
0.8103462458,
-0.286706388,
-0.1412341595,
-0.1363693923,
0.1648871154,
-0.118522875,
-0.1748315692,
-0.1090284064,
-0.1780402362,
-0.0861641839,
-0.0919658393,
0.1850931495,
0.1498368829,
-0.030898815,
0.2067725509,
-0.0318867527,
-0.2017472088,
-0.3404050171,
0.3621703088,
0.1624515951,
-0.1593184918,
0.0908347219,
0.2921066284,
-0.3177048266,
0.1487345845,
0.5308632255,
0.2546337843,
0.0195456408,
-0.2139033824,
0.0672455654,
0.4024710357,
0.0209687594,
-0.1070489064,
0.1096000746,
0.2688018382,
-0.4691239893,
-0.0098234965,
-0.0169433337,
-0.051917363,
0.3932631612,
-0.1417685002,
0.2539663315,
-0.1621345878,
0.3582160771,
-0.1994126588,
0.1481001973,
0.1612879783,
-0.0726238117,
-0.6035969853,
0.2233772278,
-0.026232779,
0.0818554163,
0.1133689657,
-0.1424365044,
-0.0047132843,
-0.0867538825,
0.138246417,
0.5117946863,
-0.0139972633,
-0.4154542089,
-0.2745565474,
-0.6609950066,
0.3881947398,
0.0333932117,
0.3113513291,
0.0009761086,
0.2808101475,
-0.0223054569,
-0.0369713232,
0.2481423765,
0.0788997263,
-0.0846414492,
0.1082659513,
-0.4920293689,
0.0982804149,
0.0012782736,
-0.1708384305,
0.0341423899,
0.2196373641,
0.0084803831,
-0.1435923874,
-0.0773816705,
0.2027597278,
-0.1723477989,
-0.0357821994,
0.2105973363,
0.4661997557,
0.0523874164,
0.0963564888,
0.0934224352,
-0.1339042783,
-0.2228315771,
-0.0566934347,
-0.0889867023,
-0.0846367776,
-0.3063417971,
0.4797859788,
-0.557139039,
-0.5037090778,
-0.3182577193,
0.2528632581,
0.0200785827,
-0.2603739202,
-0.2814156413,
0.3865294456,
-0.1927478015,
0.1288113892,
0.2811795771,
0.3434960842,
0.1242487729,
0.3990006149,
-0.4151056409,
-0.151077956,
0.7318444252,
-0.4763929844,
0.3372200727,
-0.1236958578,
0.362672627,
0.4212173522,
-0.3103901744,
-0.2815276086,
-0.0851890594,
0.1817667186,
-0.05120885,
-0.1511386782,
0.093348071,
-0.2861335278,
0.0352644399,
-0.0865419731,
0.1908098459,
0.2411005497,
-0.1176286191,
0.0448562875,
-0.0937293619
]
|
https://github.com/huggingface/datasets/issues/1942 | [experiment] missing default_experiment-1-0.arrow | When you're using metrics in a distributed setup, there are two cases:
1. you're doing two completely different experiments (two evaluations) and the 2 metrics jobs have nothing to do with each other
2. you're doing one experiment (one evaluation) but use multiple processes to feed the data to the metric.
In case 1. you just need to provide two different `experiment_id` so that the metrics don't collide.
In case 2. they must have the same experiment_id (or use the default one), but in this case you also need to provide the `num_processes` and `process_id`
If understand correctly you're in situation 2.
If so, you make sure that you instantiate the metrics with both the right `num_processes` and `process_id` parameters ?
If they're not set, then the cache files of the two metrics collide it can cause issues. For example if one metric finishes before the other, then the cache file is deleted and the other metric gets a FileNotFoundError
There's more information in the [documentation](https://huggingface.co/docs/datasets/loading_metrics.html#distributed-setups) if you want
Hope that helps ! | the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you. | 173 | [experiment] missing default_experiment-1-0.arrow
the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you.
When you're using metrics in a distributed setup, there are two cases:
1. you're doing two completely different experiments (two evaluations) and the 2 metrics jobs have nothing to do with each other
2. you're doing one experiment (one evaluation) but use multiple processes to feed the data to the metric.
In case 1. you just need to provide two different `experiment_id` so that the metrics don't collide.
In case 2. they must have the same experiment_id (or use the default one), but in this case you also need to provide the `num_processes` and `process_id`
If understand correctly you're in situation 2.
If so, you make sure that you instantiate the metrics with both the right `num_processes` and `process_id` parameters ?
If they're not set, then the cache files of the two metrics collide it can cause issues. For example if one metric finishes before the other, then the cache file is deleted and the other metric gets a FileNotFoundError
There's more information in the [documentation](https://huggingface.co/docs/datasets/loading_metrics.html#distributed-setups) if you want
Hope that helps ! | [
-0.0598983616,
0.0068150586,
0.0844844058,
0.1580612361,
0.0199415423,
-0.0320761651,
0.2774343193,
0.2207649201,
0.3088776767,
0.1602412909,
0.0177520085,
0.1031416059,
-0.2257235497,
-0.0206121989,
-0.0592387803,
-0.0310600009,
-0.0435302258,
-0.0667763725,
-0.3691175282,
-0.1325117797,
-0.3067209721,
0.5019860864,
-0.0801183507,
0.0445249565,
-0.4807969332,
0.12781322,
-0.1756108254,
0.2225742638,
-0.0789249986,
-0.5587315559,
0.377754271,
0.1831187457,
0.0421271585,
0.6000637412,
-0.0001176384,
-0.1433919519,
0.192730099,
-0.1245241538,
-0.0832809955,
-0.1930168867,
0.0741739571,
-0.3329314291,
0.4511273801,
-0.2442206591,
0.0344929099,
-0.0511981361,
0.0247722436,
-0.3721567094,
0.427414,
-0.0064347982,
0.1307448745,
0.3117668629,
-0.0682269186,
-0.3215267956,
-0.0999849588,
-0.1143520102,
0.0776159391,
0.4171808064,
-0.1312241256,
0.0894627497,
-0.274232775,
0.1245766506,
0.2123536468,
0.0292276163,
0.2388043553,
0.0246072952,
-0.0142717846,
-0.1256717891,
-0.0685604066,
0.2887805402,
0.1676788926,
-0.1594266891,
-0.2014688253,
0.0085321823,
-0.228942588,
-0.4411577582,
0.1714064032,
-0.1245808005,
0.0746697858,
0.103214547,
-0.0762278065,
-0.1549823731,
-0.0232047904,
0.1175038889,
-0.1456236988,
0.0324369334,
-0.1697128117,
0.1212586761,
-0.0279216245,
0.1497504413,
-0.3855253756,
0.3516911268,
-0.0194255896,
0.0714745671,
-0.3793984354,
0.1364801377,
0.2671203017,
0.0217041653,
0.1065051854,
0.4761020839,
-0.0047421586,
-0.0132047357,
0.3891665339,
0.173716709,
-0.064498812,
0.5233273506,
0.2027725875,
0.0692859665,
0.2185040265,
0.2179415375,
-0.1748855561,
-0.3413078785,
0.2836267352,
-0.3860960603,
0.3315580189,
0.1842790097,
0.0930347443,
-0.486887753,
0.0585247092,
0.1844706237,
-0.0363664031,
-0.1577914357,
0.0875825435,
0.3153523207,
0.1501621157,
0.0135481739,
0.2400888503,
0.3981121778,
-0.2120677233,
0.078081958,
-0.3880886436,
-0.1634973586,
-0.1441083103,
0.4293541312,
0.0517112724,
-0.1105698943,
0.685690701,
-0.1622717828,
0.4642174542,
-0.061395891,
0.1908001304,
0.1755945235,
0.0188384298,
0.2674922347,
0.0719170347,
0.1758927703,
0.2669176161,
0.1048621312,
-0.2120066881,
-0.3701926172,
-0.188737154,
-0.5549440384,
0.1263831109,
0.0321530141,
-0.4145854712,
0.2830304205,
0.3431289196,
0.2120161355,
-0.0794250742,
-0.2337054312,
0.1338795573,
0.0511646681,
-0.0858734548,
-0.1279809326,
0.4915291369,
0.7377229333,
-0.2220460325,
-0.3446712792,
0.0168372802,
-0.0487126857,
-0.0864721462,
0.3050108254,
-0.0926363692,
0.2284962684,
-0.2772684395,
0.1222093552,
0.3515836,
-0.4234383702,
-0.4113767445,
0.0080032097,
-0.4272921085,
-0.1334396005,
0.1083737835,
-0.0730171502,
0.2581317723,
0.130846858,
0.0548111387,
-0.1267486066,
0.1568038613,
-0.2836755216,
-0.3865195513,
-0.1858030409,
-0.0045480062,
-0.1014782116,
0.3006922007,
0.0563221239,
0.0986362323,
0.0875675902,
0.0597035177,
0.1062119231,
0.2606078386,
0.2477436662,
0.2262082547,
0.0003543147,
0.2899068594,
0.1102006361,
-0.1867313236,
0.2562483251,
-0.3439136744,
0.1210736111,
0.1683988422,
-0.0155255022,
-0.200071305,
-0.2549755275,
-0.1508955508,
-0.4215328097,
0.0472919159,
-0.0709692314,
0.3166151047,
0.1520329714,
-0.1438019872,
0.0916490182,
0.1583402157,
0.1830409616,
-0.4161329269,
0.077038601,
-0.0725937039,
-0.3368438184,
-0.0147533556,
0.0729851201,
0.0808982253,
0.0420650356,
-0.181738764,
0.4776446223,
-0.2574470937,
0.2789972723,
0.3836798966,
0.1822809279,
-0.0755047947,
-0.3026511073,
0.0588994548,
0.0483251475,
-0.0166238733,
0.0011706598,
-0.1728835255,
0.359136641,
0.0592463575,
0.0952931568,
-0.3097137511,
0.3031126559,
-0.0461057685,
-0.1465945989,
-0.4951241016,
-0.1190969571,
0.3968290985,
-0.3081519008,
0.2047467679,
-0.1520290077,
0.0571073815,
0.0319927409,
0.1416763216,
0.1343100071,
0.1265489608,
-0.0061656749,
-0.1617844403,
-0.0100208735,
-0.0072230622,
-0.1396546215,
0.5526064634,
0.1933411658,
0.212741226,
0.1210232824,
0.0394826531,
-0.2031922638,
0.0009545198,
0.0007294565,
-0.1463173628,
0.1554468721,
0.2229652852,
0.1337160766,
-0.2336519063,
-0.0772268176,
-0.3163038492,
0.0065657916,
-0.3755757511,
0.0749983937,
-0.1550011039,
0.4108362198,
0.0599404909,
0.0543516055,
-0.2262229919,
-0.2606705427,
0.2092503607,
-0.1175968125,
-0.0695596188,
0.3137815297,
-0.183540374,
0.5583070517,
0.017309051,
-0.0202202741,
-0.2246251106,
-0.2112009823,
-0.0854688734,
-0.148357302,
-0.1897707582,
0.232305482,
0.1300980896,
-0.1664832383,
0.0585787147,
-0.0695104301,
-0.3264134824,
0.0377148688,
0.0303656682,
0.6761792302,
0.2347931117,
0.0001521274,
-0.2518472373,
0.0283684414,
0.486887306,
-0.4140203297,
-0.2675406337,
0.0591723882,
0.0195234921,
0.0564182438,
-0.2959035635,
-0.3545248508,
0.0704141781,
-0.3689338267,
0.5647743344,
0.007240308,
-0.0687928125,
-0.2838419974,
-0.088329263,
0.1034515277,
-0.2374041975,
0.2703513205,
-0.3315574825,
-0.7032840252,
0.3768457472,
-0.3030437231,
-0.2822327018,
-0.0451200083,
0.0785692409,
0.4289897382,
-0.062586993,
-0.5393748283,
-0.4895914197,
0.0535352342,
0.1895359159,
-0.0105359759,
0.1493694186,
0.5207620263,
-0.1010605022,
-0.0189074557,
-0.078348048,
-0.2318593711,
0.6520734429,
-0.0872170106,
0.1458655298,
-0.1006414071,
0.4167236984,
0.0971857831,
0.8765501976,
0.5474239588,
0.2963705659,
0.2949185967,
0.1211512983,
0.3381682634,
-0.026252199,
-0.1907775402,
0.4884674549,
0.2340404987,
-0.1446777582,
0.2166246623,
0.1231645048,
0.1313862503,
-0.1734934151,
-0.092673324,
-0.3810845017,
-0.3277293444,
-0.1055308282,
-0.1176435351,
0.2585912943,
-0.022361597,
-0.0434566364,
-0.2274353355,
0.0631559938,
0.4233259261,
0.3457273245,
0.2006038576,
0.2726466954,
-0.1781520993,
0.0196411591,
-0.5355622172,
0.3346013725,
-0.4541781247,
0.0489894673,
-0.2220094353,
-0.243272841,
0.1035626084,
0.0479664207,
0.542753756,
0.0469459705,
-0.1899243146,
-0.0557611883,
-0.2372990996,
-0.444370538,
-0.1240650192,
-0.0472362004,
-0.0626908839,
0.2470573634,
0.3423605263,
-0.5057762861,
-0.1438982934,
-0.1255823821,
-0.1004823297,
-0.3064891696,
-0.3933879137,
-0.3682079613,
-0.0740961507,
-0.2561748326,
0.1216926351,
-0.2380999327,
0.0357329473,
-0.020143928,
0.0438734703,
-0.1827233136,
-0.0074397926,
-0.0261029191,
0.1901343912,
0.3149788678,
-0.351506263,
0.2579290271,
0.5438993573,
-0.0235522389,
0.0557700209,
0.4056895375,
0.0491007455,
-0.0081695495,
0.2634594738,
-0.0709713027,
0.287120223,
0.5680272579,
-0.2031904459,
0.0198511966,
-0.044409208,
0.4116784334,
-0.1413418651,
-0.1564922333,
0.2634869516,
-0.1303114295,
0.3417812288,
0.204519704,
0.0988922715,
0.2688886821,
-0.1753827929,
0.2153659165,
-0.1017575786,
-0.134154886,
0.3826419413,
0.0164262485,
0.9436194897,
0.205409646,
0.0592284426,
0.1934205592,
0.1771515161,
0.3641623259,
0.087618731,
0.032888487,
-0.205555886,
-0.2237053961,
0.0278851502,
-0.120230481,
-0.0501211174,
-0.2236299068,
-0.25450477,
0.4385684729,
-0.2676756084,
-0.2376936823,
-0.3451876044,
-0.1525629759,
-0.2159318775,
-0.2082404792,
0.0347567387,
0.0756388456,
0.0542349219,
0.5348837376,
-0.2138371319,
-0.1893493384,
-0.3338543773,
-0.3082467914,
-0.0714990869,
-0.0873032436,
-0.1067770869,
0.0069391062,
0.3344349563,
-0.0596456937,
-0.2383835763,
0.2095322013,
0.4598329663,
0.0625679195,
-0.2789724469,
-0.0697870925,
0.0656182319,
0.0188158769,
0.0810706466,
-0.072434105,
0.2408836633,
-0.1024616286,
-0.1515271664,
0.0943488777,
-0.1460158974,
0.0819112733,
0.0776298717,
-0.1399123818,
-0.1492316276,
-0.0896360651,
0.0508768819,
0.1414058506,
0.0980149582,
-0.2040064484,
0.1001997143,
0.2666704357,
-0.1922358125,
0.1376900077,
0.1894951612,
-0.2131110877,
-0.1155994684,
0.5188006759,
-0.2788775861,
-0.0439729802,
0.303727597,
-0.0226097256,
-0.0551310591,
-0.0629000366,
0.0001368652,
0.464610219,
-0.8037573695,
0.0456758179,
0.0220150817,
0.0338212885,
0.0662961975,
0.2331279069,
0.4397280216,
-0.1197045445,
-0.0598187372,
-0.2463521063,
-0.3375788033,
0.307607919,
-0.3285466135,
0.1088332459,
-0.3543595076,
0.1391604543,
-0.1507174224,
0.352865696,
-0.3035136759,
0.0882371441,
-0.2990915179,
-0.0992692038,
-0.0445942804,
-0.0650524497,
0.2502368689,
0.0224684626,
-0.0775944293,
0.0911417454,
-0.3674953282,
-0.1012230217,
-0.2215257436,
0.1403223127,
-0.052747108,
0.037160974,
-0.0516503043,
0.1735046357,
-0.001184576,
-0.169757247,
0.0934676304,
0.2170433104,
-0.0037537399,
0.0508842617,
0.1405667961,
0.0473802276,
-0.1137003377,
0.2166001052,
0.1527903676,
-0.2643913925,
-0.1714895666,
0.0890155137,
-0.3603460491,
-0.0957005024,
0.2447438538,
0.0772196501,
-0.1432611346,
0.2082004547,
0.0476753972,
0.0165244453,
-0.2506273985,
0.00971503,
0.4669167697,
0.1286636293,
-0.1391878873,
0.0958867744,
0.0040333066,
0.1172939539,
-0.0484066159,
0.3132458031,
0.0363496281,
0.3366865516,
0.0685618818,
0.2757846713,
0.237104848,
-0.1068967208,
0.1015352458,
0.2575311959,
0.0356711708,
-0.1651240736,
0.2285100371,
-0.1312107146,
0.0824567378,
0.3728129864,
0.6446865201,
0.1242340207,
0.2143915892,
-0.1020229831,
-0.0754536912,
0.2085905969,
-0.1400792748,
0.3282518685,
-0.158253476,
-0.1204890683,
-0.1251695305,
-0.0651013628,
0.0011577483,
-0.2720160782,
-0.3687117994,
0.6771067977,
-0.3241758645,
-0.3469700813,
-0.1790504307,
0.3376558125,
-0.145615235,
-0.1069583893,
-0.2152797431,
-0.1884309053,
-0.1496010572,
-0.1087998971,
0.2406613231,
0.0445788987,
0.2287879288,
0.0562943518,
0.016416885,
-0.2694600224,
-0.4721543193,
0.2357539684,
0.1594559252,
-0.1011681035,
0.0534250401,
0.2343873084,
-0.3595522642,
0.1360554993,
0.7672565579,
0.2582758069,
0.0755952969,
-0.0845423043,
0.0233777817,
0.2670138776,
0.0479374416,
-0.1087182984,
0.137979418,
0.1853432059,
-0.498488754,
0.0855315998,
0.1060677245,
-0.1725194752,
0.399527669,
-0.3463376462,
0.3304550052,
-0.1933651716,
0.4167953432,
-0.1988384128,
0.2309958488,
0.029862836,
-0.2229299545,
-0.7316352129,
0.3039991856,
-0.1173730344,
-0.0747347474,
0.1165495738,
-0.3806370199,
0.0477005094,
-0.0271401666,
0.1896597147,
0.3733299077,
0.0494937114,
-0.3342293501,
-0.256819129,
-0.5867592692,
0.3230749667,
0.1014964655,
0.3235938847,
-0.0898162872,
0.3570634425,
-0.0974761248,
0.137683928,
0.1949174404,
0.1135398373,
-0.10296119,
0.0712878928,
-0.4963089824,
0.1593200415,
-0.1107424423,
-0.1466664672,
0.1599318981,
0.284537077,
-0.0483199731,
-0.2016772926,
0.0270368233,
0.2672989666,
-0.1220199093,
-0.1107842848,
0.1535864174,
0.3878782094,
0.2212018222,
0.1139353067,
0.0036389539,
0.0192444026,
-0.2425038368,
-0.1357332915,
-0.1199789941,
-0.1062791198,
-0.2875246108,
0.3848076463,
-0.3711642921,
-0.3915481567,
-0.3861627281,
0.2148723304,
0.0746118501,
-0.1054602563,
-0.112957634,
0.301743567,
-0.0959621444,
-0.0575609542,
0.2854054868,
0.3148619235,
0.001485731,
0.4434119761,
-0.2427062839,
-0.1596810073,
0.7152580023,
-0.4597455263,
0.2641887367,
-0.2539712191,
0.393712759,
0.3925568163,
-0.4172927141,
-0.328201592,
0.0121976221,
0.060519591,
0.0799088702,
-0.1610700637,
0.0920377448,
-0.3906293511,
-0.1147466451,
-0.0314644352,
0.1399296075,
0.2236699313,
-0.0557529218,
0.095748879,
-0.0571785048
]
|
https://github.com/huggingface/datasets/issues/1942 | [experiment] missing default_experiment-1-0.arrow | Thank you for explaining that in a great way, @lhoestq
So the bottom line is that the `transformers` examples are broken since they don't do any of that. At least `run_seq2seq.py` just does `metric = load_metric(metric_name)`
What test would you recommend to reliably reproduce this bug in `examples/seq2seq/run_seq2seq.py`? | the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you. | 48 | [experiment] missing default_experiment-1-0.arrow
the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you.
Thank you for explaining that in a great way, @lhoestq
So the bottom line is that the `transformers` examples are broken since they don't do any of that. At least `run_seq2seq.py` just does `metric = load_metric(metric_name)`
What test would you recommend to reliably reproduce this bug in `examples/seq2seq/run_seq2seq.py`? | [
0.0750926808,
-0.1511458308,
0.1568089426,
0.1809433997,
-0.0645125136,
-0.0121278428,
0.3743320704,
0.2074545026,
0.2033591121,
0.0637142807,
0.2323808074,
0.1092877761,
-0.3682079613,
-0.2463027239,
0.1191040277,
-0.159807235,
0.0618004128,
0.0787237734,
-0.398302108,
-0.1459855586,
-0.2288622707,
0.597700417,
-0.2141059339,
0.0435038023,
-0.5726749897,
0.2785583735,
-0.2246072888,
0.1813749671,
0.1039817408,
-0.5100976229,
0.4914011061,
0.1075302884,
-0.0393091589,
0.6184600592,
-0.0001310607,
-0.1159783155,
0.2905943692,
-0.2518159449,
-0.1454311758,
-0.2269470841,
-0.158411324,
-0.2461212873,
0.449108988,
-0.2053941488,
0.0618129633,
0.0542068183,
0.1260378212,
-0.1677698195,
0.4823169112,
0.03672795,
0.0278775431,
0.4897831082,
-0.1625122428,
-0.3433549702,
0.0686697289,
0.1456457675,
0.0055332943,
0.2566857338,
-0.0682887435,
0.0118006421,
-0.2210908085,
0.2391413152,
0.2916742563,
-0.0231564324,
0.2404491603,
0.0032987427,
-0.0292709991,
-0.0683817118,
-0.1375892013,
0.3957450986,
0.2470199764,
-0.1506507993,
-0.3314957619,
-0.0105180657,
-0.3707769513,
-0.193893522,
0.3266066015,
-0.1777870804,
0.0734512433,
0.1111948043,
-0.0882411823,
-0.1193778738,
-0.0133478353,
0.0675631762,
-0.2427281886,
0.0775501877,
-0.0889167115,
0.0202898663,
-0.0826741382,
0.0575082153,
-0.2245982289,
0.2535659969,
-0.0392757244,
0.1483732164,
-0.3013094068,
-0.0075874068,
0.1568769962,
-0.09263587,
0.0377057977,
0.399567306,
-0.0197047889,
0.1337979138,
0.3218588829,
0.1455345601,
-0.0883823931,
0.5639247298,
0.2473169267,
0.1956647336,
0.1423612982,
0.2767653465,
-0.0769011155,
-0.2230590731,
0.1054571122,
-0.1692463607,
0.3477956355,
0.064889662,
0.0919377357,
-0.4264973402,
0.1201482862,
0.2431014627,
-0.0429431275,
-0.1472579837,
0.1478134394,
0.4604138136,
0.1981286407,
0.0761527792,
0.1781303734,
0.3603521585,
-0.1025499552,
-0.0604588129,
-0.3435696661,
-0.1752092838,
-0.2113111466,
0.2515897155,
0.2330030203,
0.1246439219,
0.6372714639,
-0.246567145,
0.3610938191,
-0.1116093099,
0.3067768812,
0.2852131128,
0.1577806324,
0.4128850996,
-0.1351287663,
0.2474036962,
0.3334743083,
0.0782881901,
-0.2614817619,
-0.1910318434,
-0.1088008583,
-0.4993452728,
0.2095869631,
-0.0593760572,
-0.6320043802,
0.2099976689,
0.3444992602,
0.1252348572,
-0.0880120471,
-0.2906295955,
0.1899116784,
0.0113040665,
0.0194317326,
0.0363645442,
0.5021128654,
0.8084044456,
-0.1071280614,
-0.5354681015,
-0.0404282808,
0.0006154198,
-0.0503947288,
0.1723444611,
-0.0216382705,
0.1558131725,
-0.2820473015,
0.0270248055,
0.3047231138,
-0.3449213803,
-0.3754405379,
-0.0424834341,
-0.2123758793,
-0.0130928326,
0.1726595163,
-0.0992882177,
0.1596010774,
0.0654160082,
0.0390035361,
-0.1574851125,
0.1784498692,
-0.1477154642,
-0.2838847339,
-0.3624430299,
0.0209673066,
-0.0074380105,
0.3962643147,
0.1487721354,
-0.0420771688,
0.2471258044,
-0.0140904253,
0.1546951532,
0.1208310947,
0.2546757758,
0.1531661749,
-0.2394437045,
0.3301899731,
-0.0040532332,
-0.3316980004,
0.3137979209,
-0.4128646851,
-0.0016716997,
0.1717611998,
0.0299319439,
-0.3266587853,
-0.1333885491,
-0.055923745,
-0.4057340622,
-0.0640613362,
-0.06816753,
0.1189895272,
0.0956796631,
-0.1376005709,
0.0342137963,
0.2938392758,
0.2461945564,
-0.4998992085,
0.084135294,
0.0144147053,
-0.2128110677,
-0.2256051451,
0.0138928536,
-0.0110580204,
-0.1588858664,
-0.2033100128,
0.369086206,
-0.06673529,
0.3717398942,
0.2778243721,
0.3218530416,
0.0117865223,
-0.4765184224,
-0.0597841665,
0.2749159038,
-0.0537503958,
-0.0168478619,
-0.456501931,
0.3429724574,
0.1099388078,
0.0574721359,
-0.2757982612,
0.3847723901,
-0.036700923,
-0.2347463667,
-0.5471624732,
-0.1155280322,
0.4180022776,
-0.2595299482,
0.2554793656,
-0.1064639464,
0.1143486425,
-0.2071105242,
0.136657685,
-0.0392938815,
0.2872010469,
0.1524625868,
-0.3554167747,
-0.061928656,
0.0335069522,
-0.0804225355,
0.3691935241,
0.0703881532,
0.2867378294,
-0.0314373635,
0.0858655199,
-0.1533984393,
0.168610245,
0.1308804899,
-0.118894361,
0.1236362681,
0.212359935,
0.1744193137,
-0.2839015126,
-0.1667080969,
-0.2365746796,
0.0640982017,
-0.3988456726,
0.1490960568,
-0.0114228353,
0.5295212269,
0.0435040668,
-0.0604520217,
-0.2244166434,
-0.2906612754,
0.1571871042,
-0.2209061831,
-0.0536586717,
0.3262692094,
-0.2227447331,
0.6643275619,
0.0525770523,
-0.186557129,
-0.4059664011,
-0.0892627761,
-0.1126824319,
-0.1945126504,
-0.262727052,
0.0734462291,
-0.1226369962,
-0.1925716251,
0.0805477127,
0.1065049767,
-0.4834922254,
0.1850723326,
0.2666203082,
0.6751853228,
0.3146784008,
-0.0235571321,
-0.1379267126,
-0.0140280686,
0.3056757748,
-0.4094472528,
-0.243708849,
0.3083648682,
-0.0299442913,
0.1579079032,
-0.2975550592,
-0.2534160316,
0.1630840749,
-0.259406656,
0.5369833112,
0.0357926078,
-0.0390027948,
0.0486772694,
-0.0482488312,
0.1972009689,
-0.2278416902,
0.2416344136,
-0.0970919356,
-0.4955852032,
0.4939000607,
-0.089977093,
-0.2325499803,
-0.2330011427,
-0.2596038282,
0.2908663154,
-0.0499921143,
-0.4904942811,
-0.2846312523,
-0.155435726,
0.10770078,
0.0514278673,
0.0468783341,
0.5173731446,
-0.0740087405,
0.0814282522,
-0.1966247708,
-0.1054341421,
0.4829237163,
-0.0403455719,
0.2072459608,
-0.1482864469,
0.2523772418,
0.1457010806,
0.9683125615,
0.4021626413,
0.2108837962,
0.1378670633,
0.1212734655,
0.2691401243,
-0.0327656865,
-0.1612856537,
0.129048124,
0.1736799181,
-0.2389680296,
0.1625218987,
0.1619472653,
0.0524046943,
-0.0170533396,
0.048059497,
-0.3372258544,
-0.3736347854,
0.0258930679,
-0.2867442667,
0.2674738467,
0.0084653348,
0.12985228,
-0.2105973959,
0.0496195219,
0.3940188587,
0.3825773895,
0.2130385339,
0.1041272953,
-0.1515609026,
0.1164902672,
-0.4340968728,
0.1125115231,
-0.4435681105,
0.2958868742,
-0.1493598968,
-0.3472633362,
-0.0203617848,
-0.0741199255,
0.7864436507,
0.1294676065,
-0.01218371,
-0.0056255446,
-0.1805306822,
-0.527991116,
-0.1744876355,
-0.2424482852,
-0.0621801317,
0.3286207914,
0.4173187315,
-0.3465722799,
-0.0914421678,
-0.0846181735,
-0.1157487482,
-0.257594347,
-0.1866010427,
-0.3307994008,
0.0978285521,
-0.1791492552,
0.1057737768,
-0.1725384146,
-0.1363508403,
0.0036878069,
0.1023928449,
-0.1907084584,
-0.0807409212,
0.0790345669,
0.3291590512,
0.3670136929,
-0.4262427986,
0.2056542188,
0.5175887346,
-0.3233254254,
-0.137478739,
0.3022693396,
0.147537291,
-0.1070955172,
0.4146630466,
-0.0502336957,
0.1603398025,
0.5822023153,
-0.2052156478,
-0.0157066993,
0.0857769549,
0.3517509401,
-0.1212828383,
-0.0598318949,
0.2842307985,
-0.1013545617,
0.2932532728,
0.2780108154,
0.4115168452,
0.201475516,
-0.2698431611,
0.2848834097,
0.0203257501,
-0.1558413804,
0.5100289583,
0.0391435511,
1.0379651785,
0.154809162,
0.201515004,
0.1303993016,
0.1648874879,
0.4351430535,
0.1203606725,
0.0437970608,
-0.2623808086,
-0.3548330069,
-0.0181118809,
-0.2812708318,
-0.0030667426,
-0.1445854008,
-0.4378489554,
0.3533286154,
-0.3426380157,
0.0178091731,
-0.0403171331,
-0.2422981709,
-0.2960455716,
-0.1201757491,
0.1541962177,
-0.0456661768,
0.1980381012,
0.6490918994,
-0.2589023709,
-0.1568100303,
-0.3486699164,
-0.412861675,
-0.2985282242,
0.0445989147,
-0.0711565688,
-0.0084003694,
0.560911715,
-0.088436313,
-0.050209634,
0.2643804252,
0.4029279947,
0.1458511651,
-0.4677397013,
0.1415467858,
-0.0590567179,
0.1639375091,
0.1763227433,
0.0302407034,
0.1974539906,
0.0159926564,
-0.0811610073,
0.0317444429,
-0.2748937309,
0.1612438709,
0.0895967111,
-0.1340848505,
-0.2504482865,
-0.0825361535,
0.0357696339,
0.1338221282,
-0.0820519701,
-0.2147387266,
0.0269698836,
0.2467704713,
-0.2292546928,
0.0371178873,
0.1516253203,
-0.2628641427,
-0.0579270348,
0.4928118587,
-0.3625270128,
-0.0921284035,
0.3880808651,
-0.0145979561,
0.0954933092,
-0.0928230733,
0.1040696129,
0.4269272089,
-0.9760486484,
0.1369952261,
-0.3507161736,
-0.186986059,
0.0663212389,
0.2257799059,
0.6225937605,
-0.1898289919,
-0.1334912032,
-0.1952226609,
-0.3736695051,
0.3917948902,
-0.1390204877,
0.1900529116,
-0.5208064914,
-0.0400966592,
-0.0909904167,
0.2423183322,
-0.1821932495,
0.2231492102,
-0.2955842912,
-0.0602546036,
-0.1686765701,
-0.0956605077,
0.3259902298,
-0.1957740784,
-0.1448484808,
-0.0011049631,
-0.3109073639,
-0.0424051434,
-0.3904663324,
0.1731739193,
-0.0693072379,
0.0203192309,
-0.1117977574,
0.4331069589,
0.042607002,
-0.2253695875,
0.0421759188,
0.0808039755,
0.0257404707,
0.296879977,
0.1680520773,
0.07123027,
-0.2817443907,
0.088296622,
0.1818268299,
-0.1884133071,
0.0025737747,
0.1377199888,
-0.3446249962,
-0.0645115227,
0.0776906386,
0.2567933798,
-0.1901201308,
0.0443107784,
0.1996379495,
-0.0354052521,
-0.1188759208,
-0.055423364,
0.3305152953,
0.1367083043,
-0.1593907624,
0.0950240791,
-0.0886048153,
0.0568299107,
-0.0802779272,
0.1048179567,
0.0006988937,
0.3218780458,
0.0853814706,
0.3245590031,
0.1636348516,
-0.1622795016,
0.3574334085,
0.1928836554,
0.1937336177,
-0.1373786479,
0.3820770979,
0.0233727768,
-0.0269825477,
0.4478303194,
0.451541543,
-0.0703796074,
0.2798922658,
-0.1402745843,
0.0753537342,
0.2244552523,
-0.2527287602,
0.3090750873,
-0.0772220567,
-0.1782063842,
-0.1137574539,
0.0177020002,
0.0819642693,
0.0149575369,
-0.2574416995,
0.5996486545,
-0.3609504402,
-0.1359238923,
-0.305848062,
0.0015198162,
0.0109868487,
-0.2694583833,
-0.0101672467,
-0.1457813829,
-0.2194042653,
-0.2321440428,
0.2223371714,
0.0849189237,
0.0927912146,
0.0869171247,
0.0281846728,
-0.1800114959,
-0.2946359515,
0.3112851679,
0.2292451859,
-0.2502250671,
0.1192080528,
0.2937875986,
-0.3188092709,
0.0803372115,
0.6347106695,
0.2948593497,
-0.0884072706,
-0.0761147067,
0.1265600175,
0.1849356741,
-0.0109213283,
-0.0974582508,
0.2389877737,
0.1733165681,
-0.3825125396,
-0.0470268317,
0.0349704549,
-0.1180281192,
0.336517036,
-0.217898041,
0.1758397967,
-0.2750439644,
0.5571628213,
-0.2242749035,
0.0447614416,
0.1200993136,
-0.1157298908,
-0.604126215,
0.2573816478,
-0.1305812597,
-0.0253196079,
0.123965688,
-0.0469637513,
-0.0171992201,
0.0293213725,
0.2097138017,
0.4323585033,
0.2327562571,
-0.2703853548,
-0.2339158058,
-0.5324943662,
0.2602302134,
0.1255425364,
0.3936371505,
0.2322482318,
0.2296852469,
-0.0150975492,
-0.0309842583,
0.108906813,
-0.0064850356,
-0.1906320453,
0.0576797239,
-0.449859947,
0.0906726718,
-0.0608778074,
-0.0421847254,
0.0336841829,
0.0912770852,
-0.0197496768,
-0.0872879252,
-0.0868773162,
0.1656351238,
-0.2633647025,
-0.053527467,
0.144715488,
0.3367944062,
0.1615677029,
0.0700880885,
0.0182320457,
-0.1633972973,
-0.2470240891,
-0.1918810457,
0.0415443927,
-0.2905655801,
-0.3666419089,
0.4213338494,
-0.292668134,
-0.3824874163,
-0.323888272,
0.1042758375,
0.180890739,
-0.104053095,
-0.393733561,
0.3160437346,
-0.272498548,
0.0433947407,
0.282597363,
0.3950479329,
-0.1257579327,
0.372269839,
-0.2413483262,
-0.0346615352,
0.6833052039,
-0.5341156721,
0.1998462677,
-0.336379081,
0.3415122926,
0.2691198587,
-0.3390434086,
-0.3327303231,
-0.1527007818,
0.1526988447,
-0.0082968036,
-0.1418136209,
0.0664726943,
-0.1462515891,
-0.0786666125,
-0.08019232,
0.4183966517,
0.1686917841,
-0.0924175903,
-0.0271847248,
-0.1010507867
]
|
https://github.com/huggingface/datasets/issues/1942 | [experiment] missing default_experiment-1-0.arrow | To give more context, we are just using the metrics for the `comput_metric` function and nothing else. Is there something else we can use that just applies the function to the full arrays of predictions and labels? Because that's all we need, all the gathering has already been done because the datasets Metric multiprocessing relies on file storage and thus does not work in a multi-node distributed setup (whereas the Trainer does).
Otherwise, we'll have to switch to something else to compute the metrics :-( | the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you. | 85 | [experiment] missing default_experiment-1-0.arrow
the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you.
To give more context, we are just using the metrics for the `comput_metric` function and nothing else. Is there something else we can use that just applies the function to the full arrays of predictions and labels? Because that's all we need, all the gathering has already been done because the datasets Metric multiprocessing relies on file storage and thus does not work in a multi-node distributed setup (whereas the Trainer does).
Otherwise, we'll have to switch to something else to compute the metrics :-( | [
0.0360683352,
0.0379048288,
0.0943588987,
0.1253586709,
0.1760240346,
0.05072283,
0.181993857,
0.2530151308,
0.2025466263,
0.251921773,
0.0820864663,
0.1800010353,
-0.3751124442,
0.0665907264,
0.1416407377,
-0.0772905722,
0.0167335495,
0.0033795102,
-0.3133100271,
-0.1381400973,
-0.2079615444,
0.448179394,
-0.1228018925,
0.0743640438,
-0.5695957541,
0.1498475373,
-0.1701163054,
0.0805466101,
-0.1255112141,
-0.5812903047,
0.3919238746,
0.1458095908,
0.0017236554,
0.4191245735,
-0.0001262029,
-0.1343241185,
0.1936456263,
-0.2333319038,
-0.1047415435,
-0.2225058079,
0.1268718541,
-0.3101156652,
0.522464931,
-0.1460826397,
-0.0495460816,
-0.0838489607,
0.0543664023,
-0.4214366078,
0.4781625569,
0.118394427,
0.0403270312,
0.3758566082,
0.0349312611,
-0.2492557317,
-0.0176794697,
-0.0774545372,
0.0102453623,
0.3299077749,
-0.0517303906,
-0.0297791176,
-0.2794108093,
0.2436844558,
0.2794157267,
-0.0088509265,
0.3128021955,
-0.0297788139,
-0.0145230526,
-0.1737940758,
-0.1312472373,
0.2608570158,
0.1949307919,
-0.208975777,
-0.3556881249,
-0.0132944202,
-0.0411425717,
-0.5314990282,
0.1682945639,
-0.1075423807,
-0.0336156972,
0.0474930331,
-0.1316338778,
-0.1672554016,
-0.0719778463,
0.0874652714,
-0.1760179102,
0.0398987979,
-0.1421071142,
0.0187714323,
0.0959143564,
0.2716264427,
-0.2097330838,
0.4107066393,
0.1014251634,
0.1463737488,
-0.3606157005,
0.082555972,
0.1721962839,
-0.0282379519,
0.0502632223,
0.3950389028,
0.0560898222,
0.1017730683,
0.3755904138,
0.0602641143,
-0.0459786616,
0.4311772585,
0.2344247997,
0.1357564181,
0.116066359,
0.2224395871,
-0.1367135048,
-0.2810333669,
0.18331559,
-0.1449777633,
0.3832838535,
0.2245604843,
-0.1369668096,
-0.3769402802,
0.0637292489,
0.2464853674,
-0.0436971113,
-0.2041593194,
0.1965016276,
0.2750816941,
0.1341593564,
0.1387403458,
0.1868601888,
0.3029897809,
-0.1722472757,
-0.1656984985,
-0.3989240825,
-0.0746449232,
-0.2094952613,
0.3997787535,
0.1894152015,
-0.04119993,
0.6616483927,
-0.3590563834,
0.5086168647,
-0.2140402347,
0.1602293253,
0.1458079368,
0.097426787,
0.2898180783,
-0.0214461368,
0.099196963,
0.3203159869,
0.0583866313,
-0.1933313459,
-0.3334260583,
-0.2335584015,
-0.5762782693,
0.1160739884,
-0.0552394725,
-0.512142837,
0.2240831703,
0.1648782641,
0.3089992702,
-0.1530396193,
-0.1348858625,
0.1365174651,
-0.0361997485,
-0.1778378338,
-0.0181773547,
0.6248612404,
0.5545914769,
-0.3065956235,
-0.4664386511,
0.1092809141,
-0.0107355509,
-0.1018489376,
0.1589732021,
-0.060335651,
0.3344115615,
-0.1820727587,
0.087040633,
0.4544462264,
-0.4080087841,
-0.4083964229,
-0.253352344,
-0.229306668,
-0.1433105767,
0.0884948298,
-0.0339341201,
0.1266492456,
0.2276443839,
0.0750585571,
-0.2100954503,
0.1428104192,
-0.3406260312,
-0.229255259,
-0.2566598952,
-0.0860029757,
0.0165202338,
0.4695227444,
-0.0004948126,
-0.0502113812,
0.0669919327,
0.055291988,
0.0273391362,
0.2316126227,
0.2274737656,
0.18492046,
-0.0041500814,
0.1867881417,
-0.0504859686,
-0.2215746045,
0.3026399016,
-0.293017447,
0.0360220186,
0.1714073718,
-0.0174640529,
-0.1159895733,
-0.0948146656,
-0.0791353285,
-0.3731917441,
-0.0357884318,
-0.0878943354,
0.2329108864,
0.1419439465,
-0.2297813594,
0.1230705082,
-0.0462220535,
0.2593326569,
-0.3241709173,
0.1597559452,
-0.0609498508,
-0.3210316002,
-0.0040194453,
0.2121323198,
-0.1006769985,
0.0424050316,
-0.1941401362,
0.3698190451,
-0.143566072,
0.1763956994,
0.3263867497,
0.1948136091,
-0.0946417823,
-0.468090415,
-0.1868380457,
0.0226580892,
-0.0780185387,
-0.042573113,
-0.1915087849,
0.4211586714,
0.1166607216,
0.1690176576,
-0.2548605204,
0.3526452184,
-0.1358465552,
-0.0465469658,
-0.513710618,
-0.1119693592,
0.3400012851,
-0.4222238362,
0.3011051714,
-0.1835050881,
-0.0250692293,
-0.0542988069,
0.2423657775,
0.1083918586,
0.1255673021,
0.1039018854,
-0.2200978398,
-0.0708631575,
-0.0215852261,
-0.1932942271,
0.4366639853,
0.1965453029,
0.2774852812,
0.0860512853,
0.0942833424,
-0.1230534315,
0.0770649537,
0.1215848997,
-0.0986285061,
0.0737262666,
0.194255203,
0.1487441212,
-0.3226958215,
-0.0074755484,
-0.3011006415,
0.0195339676,
-0.3337208331,
0.0596699528,
-0.236871317,
0.3630672693,
0.1683838814,
-0.0021909331,
-0.233354792,
-0.2706064582,
0.2153630257,
-0.1962648332,
-0.0221890528,
0.3150246739,
-0.2984646261,
0.6049126983,
0.0615461245,
-0.022624813,
-0.2279760689,
-0.1495147496,
-0.0368126854,
-0.1780067682,
-0.1940799206,
0.096849218,
0.0374841504,
-0.149942562,
0.1426576972,
-0.1025386751,
-0.3601031005,
0.0933404341,
0.0195363015,
0.6119939685,
0.3463462889,
-0.0454065986,
-0.352743566,
0.0991579518,
0.4500067532,
-0.4079984128,
-0.2767030001,
0.1133302078,
0.0314445309,
0.1227025613,
-0.2230908126,
-0.2890610695,
0.0112663899,
-0.2815253437,
0.5991317034,
0.0410661288,
-0.0020706982,
-0.2288423479,
-0.0237934329,
0.1773279905,
-0.3290061057,
0.2469100058,
-0.2935865819,
-0.7149357796,
0.4781394601,
-0.0908918977,
-0.2723423541,
-0.1058706269,
0.0675314739,
0.434768647,
-0.0653752908,
-0.6561146975,
-0.4437873065,
0.0903979689,
0.0918387547,
-0.0425769985,
0.1582769454,
0.5028117299,
-0.064025417,
0.0077045066,
-0.1060190573,
-0.2926115692,
0.5380768776,
-0.1749774218,
0.1555452794,
-0.2270887941,
0.2927688062,
0.2596624792,
0.9461432099,
0.5229725838,
0.1494288594,
0.2371039987,
0.1094774306,
0.4445638359,
-0.069985874,
-0.1495136172,
0.3707888424,
0.1129483432,
-0.0162508208,
0.2179197669,
0.0888909325,
0.1427087337,
-0.1463133246,
0.0634613037,
-0.2214712501,
-0.3302626014,
-0.0235039629,
-0.1392382085,
0.1961391419,
0.0105120558,
0.116101034,
-0.2785866559,
-0.0160786752,
0.3919546604,
0.2068582624,
0.1829010248,
0.27927652,
-0.207949698,
-0.1412258446,
-0.5621308088,
0.2452996373,
-0.3804306388,
0.045309756,
-0.1028639004,
-0.2098940015,
0.1184137315,
0.0432682671,
0.4675576985,
0.0269833785,
-0.2499114722,
0.0441783071,
-0.1463384777,
-0.3195357025,
-0.1433726102,
-0.0926064923,
-0.0362703204,
0.2236896008,
0.3636892438,
-0.3087712228,
-0.2344550788,
-0.0264694691,
-0.0768965557,
-0.2622459233,
-0.2304196358,
-0.3326176703,
0.0181396417,
-0.1006646305,
0.2380574346,
-0.2081853598,
0.0290680341,
0.058160089,
0.0281243604,
-0.1481355578,
-0.0308292694,
0.0667478517,
0.1422159225,
0.2505322099,
-0.2525005937,
0.1854340583,
0.5488845706,
-0.0489845425,
0.0482747108,
0.3769326806,
-0.0075405994,
-0.0765793547,
0.2972932458,
-0.1136461273,
0.4294684231,
0.5456268787,
-0.2386383563,
-0.0288394578,
0.0150821144,
0.3863059282,
-0.1887577027,
0.0419145152,
0.3080278337,
-0.0701058656,
0.3514489233,
0.2013501227,
0.2649253607,
0.3189359307,
-0.1359727234,
0.3833770454,
0.1452752799,
-0.168472454,
0.2444074303,
0.140688628,
0.938252449,
0.0755249709,
0.1895241439,
0.1168171391,
0.1613008976,
0.5568842888,
0.0864995942,
0.0911397412,
-0.0336711891,
-0.3376880288,
-0.029873509,
-0.1386371255,
-0.056579411,
-0.2417145818,
-0.1862821579,
0.4872969091,
-0.2020583302,
-0.1370245963,
-0.1831812412,
-0.1983543187,
-0.0345439836,
-0.2534299493,
0.1010972038,
-0.0277634226,
-0.0001073712,
0.4575576484,
-0.1977292895,
-0.1139500886,
-0.275044471,
-0.3137762249,
-0.23209095,
-0.0686380863,
-0.3299610019,
-0.0637358353,
0.389051497,
-0.0742628053,
-0.0691312626,
0.2970838845,
0.4629917443,
0.0839800164,
-0.345638603,
0.023330288,
0.000266728,
0.0664639249,
0.0784113333,
0.0073579201,
0.321621716,
-0.0662751496,
0.0102047585,
0.0647310317,
-0.234732002,
0.0867331028,
0.1223053336,
-0.1585002095,
-0.0257759616,
-0.0164249465,
0.1262874901,
0.0988366455,
0.0305558257,
-0.326099813,
0.0412871465,
0.2831501365,
-0.2210922837,
0.1518936753,
0.1960546076,
-0.2191641182,
-0.0440746397,
0.5630207062,
-0.2119452953,
-0.1510917991,
0.4201503396,
-0.015550754,
-0.0242567137,
-0.0876444653,
-0.0401976369,
0.5411636829,
-0.6515187621,
0.0948462561,
-0.0788774714,
-0.0676447153,
0.024186058,
0.2146993876,
0.4476055801,
-0.1635666341,
-0.0357840508,
-0.1667280644,
-0.4597931802,
0.3591094911,
-0.2916518152,
0.1436113715,
-0.4845040143,
0.2159709781,
-0.1050218195,
0.311829865,
-0.2253036946,
0.1461491287,
-0.3180458844,
-0.1291921884,
-0.0933288932,
0.0110472841,
0.2815723419,
-0.0890989155,
-0.1196711361,
0.0586081408,
-0.3794822097,
-0.0509908199,
-0.2384001911,
0.1548077166,
-0.0630956814,
0.1762831062,
0.077498816,
0.2939428091,
-0.1219548658,
-0.202815339,
-0.0756871924,
0.1594336927,
0.0939317197,
0.1515527517,
0.0464457795,
0.1661392301,
-0.0703115687,
0.0964261442,
0.2369104475,
-0.1221290305,
-0.1685437411,
0.0712453052,
-0.2272637784,
-0.1083661392,
0.1468649954,
0.0859439299,
-0.2312788516,
0.1614070833,
0.1170421913,
0.0157427657,
-0.3260177076,
-0.0447356068,
0.4571227431,
0.151857987,
-0.1727566868,
0.1071675122,
-0.0483279824,
0.0419552885,
-0.1785604209,
0.2204388082,
0.0308614708,
0.3870634139,
0.07500121,
0.2414392233,
0.2413667291,
-0.0906782299,
0.1284104437,
0.147979334,
-0.1151853651,
-0.1851311773,
0.3271122277,
-0.0866595134,
0.1272320002,
0.2972465158,
0.5802829862,
0.1529764235,
0.1836085171,
0.026347829,
-0.1465579867,
0.2086514384,
-0.1388538182,
0.2844024599,
-0.2059946507,
-0.0926633105,
-0.2410399318,
0.0577585362,
0.0592740104,
-0.1637321115,
-0.5557779074,
0.8412663937,
-0.2907381356,
-0.3601016104,
-0.1830141693,
0.1928286552,
-0.0918844864,
-0.1444140226,
-0.1394793838,
-0.1471370459,
-0.1995597482,
-0.1521383673,
0.2422815561,
-0.0227819793,
0.2497123331,
0.0456515104,
0.0298937373,
-0.2345263511,
-0.5137044191,
0.2872683108,
0.2645597756,
-0.1797737032,
0.1278649718,
0.1644398272,
-0.3647378087,
0.1035096869,
0.7005338073,
0.2391312867,
-0.0577403456,
-0.100166887,
0.0456192344,
0.2528727353,
-0.0410718843,
-0.0698785484,
0.1960647702,
0.3556524217,
-0.5932105184,
0.1043867618,
-0.0007091698,
-0.0965856612,
0.402624011,
-0.2877337337,
0.3789044917,
-0.2412758619,
0.4647474885,
-0.1182561964,
0.044255469,
0.0336354859,
-0.1767839938,
-0.6990653872,
0.3790698349,
-0.0600341931,
0.0051058973,
0.0743903518,
-0.257763654,
0.0131136449,
-0.0343394503,
0.2901058495,
0.42056638,
0.0609132908,
-0.2994612455,
-0.2646943033,
-0.5396560431,
0.1927631497,
0.1414520741,
0.3431107104,
-0.0039900574,
0.3530116677,
0.0309118703,
0.0716794655,
0.2382591963,
-0.0196618829,
-0.060088288,
-0.0279573016,
-0.6378628016,
0.1246492639,
-0.1350912154,
0.0022303166,
0.1786824614,
0.3266236782,
0.0166259389,
-0.2267822921,
-0.0830881149,
0.3012849987,
-0.0886904001,
-0.1383719891,
0.3098230064,
0.4241037965,
0.0609220043,
0.1810895801,
0.0859954506,
-0.0158587638,
-0.1867470294,
-0.1026433334,
-0.0907716602,
-0.0847368762,
-0.279447794,
0.4343643785,
-0.4776820838,
-0.3170900047,
-0.3601941764,
0.0708862469,
0.1506472528,
-0.1097971424,
-0.3143192232,
0.3568704426,
-0.1146318167,
0.0508092418,
0.3609867394,
0.3676593006,
-0.0981446207,
0.3505347073,
-0.3764446676,
-0.1778482348,
0.7721179128,
-0.4845306277,
0.2451474816,
-0.3426679075,
0.3337126374,
0.6285011768,
-0.2959863245,
-0.5779032111,
-0.0101890536,
0.1667892486,
0.0562026538,
-0.3047440946,
0.1543696821,
-0.4789427817,
0.0177682135,
-0.0675173923,
0.3459008932,
0.113831535,
-0.0296074916,
-0.042008318,
-0.1831469536
]
|
https://github.com/huggingface/datasets/issues/1942 | [experiment] missing default_experiment-1-0.arrow | OK, it definitely leads to a race condition in how it's used right now. Here is how you can reproduce it - by injecting a random sleep time different for each process before the locks are acquired.
```
--- a/src/datasets/metric.py
+++ b/src/datasets/metric.py
@@ -348,6 +348,16 @@ class Metric(MetricInfoMixin):
elif self.process_id == 0:
# Let's acquire a lock on each node files to be sure they are finished writing
+
+ import time
+ import random
+ import os
+ pid = os.getpid()
+ random.seed(pid)
+ secs = random.randint(1, 15)
+ time.sleep(secs)
+ print(f"sleeping {secs}")
+
file_paths, filelocks = self._get_all_cache_files()
# Read the predictions and references
@@ -385,7 +395,10 @@ class Metric(MetricInfoMixin):
if predictions is not None:
self.add_batch(predictions=predictions, references=references)
+ print("FINALIZE START")
+
self._finalize()
+ print("FINALIZE END")
self.cache_file_name = None
self.filelock = None
```
then run with 2 procs: `python -m torch.distributed.launch --nproc_per_node=2`
```
export BS=16; rm -r output_dir; PYTHONPATH=src USE_TF=0 CUDA_VISIBLE_DEVICES=0,1 python -m torch.distributed.launch --nproc_per_node=2 examples/seq2seq/run_seq2seq.py --model_name_or_path t5-small --output_dir output_dir --adam_eps 1e-06 --do_eval --do_train --do_predict --evaluation_strategy=steps --label_smoothing 0.1 --learning_rate 3e-5 --logging_first_step --logging_steps 1000 --max_source_length 128 --max_target_length 128 --num_train_epochs 1 --overwrite_output_dir --per_device_eval_batch_size $BS --per_device_train_batch_size $BS --predict_with_generate --eval_steps 25000 --sortish_sampler --task translation_en_to_ro --val_max_target_length 128 --warmup_steps 500 --max_train_samples 10 --max_val_samples 10 --max_test_samples 10 --dataset_name wmt16 --dataset_config ro-en --source_prefix "translate English to Romanian: "
```
```
***** Running Evaluation *****
Num examples = 10
Batch size = 16
0%| | 0/1 [00:00<?, ?it/s]FINALIZE START
FINALIZE START
sleeping 11
FINALIZE END
100%|██████████████████████████████████████████████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:11<00:00, 11.06s/it]
sleeping 11
Traceback (most recent call last):
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/metric.py", line 368, in _finalize
self.data = Dataset(**reader.read_files([{"filename": f} for f in file_paths]))
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/arrow_reader.py", line 236, in read_files
pa_table = self._read_files(files, in_memory=in_memory)
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/arrow_reader.py", line 171, in _read_files
pa_table: pa.Table = self._get_dataset_from_filename(f_dict, in_memory=in_memory)
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/arrow_reader.py", line 302, in _get_dataset_from_filename
pa_table = ArrowReader.read_table(filename, in_memory=in_memory)
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/arrow_reader.py", line 322, in read_table
stream = stream_from(filename)
File "pyarrow/io.pxi", line 782, in pyarrow.lib.memory_map
File "pyarrow/io.pxi", line 743, in pyarrow.lib.MemoryMappedFile._open
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 97, in pyarrow.lib.check_status
FileNotFoundError: [Errno 2] Failed to open local file '/home/stas/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow'. Detail: [errno 2] No such file or directory
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "examples/seq2seq/run_seq2seq.py", line 645, in <module>
main()
File "examples/seq2seq/run_seq2seq.py", line 601, in main
metrics = trainer.evaluate(
File "/mnt/nvme1/code/huggingface/transformers-mp-pp/src/transformers/trainer_seq2seq.py", line 74, in evaluate
return super().evaluate(eval_dataset, ignore_keys=ignore_keys, metric_key_prefix=metric_key_prefix)
File "/mnt/nvme1/code/huggingface/transformers-mp-pp/src/transformers/trainer.py", line 1703, in evaluate
output = self.prediction_loop(
File "/mnt/nvme1/code/huggingface/transformers-mp-pp/src/transformers/trainer.py", line 1876, in prediction_loop
metrics = self.compute_metrics(EvalPrediction(predictions=preds, label_ids=label_ids))
File "examples/seq2seq/run_seq2seq.py", line 556, in compute_metrics
result = metric.compute(predictions=decoded_preds, references=decoded_labels)
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/metric.py", line 402, in compute
self._finalize()
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/metric.py", line 370, in _finalize
raise ValueError(
ValueError: Error in finalize: another metric instance is already using the local cache file. Please specify an experiment_id to avoid colision between distributed metric instances.
``` | the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you. | 452 | [experiment] missing default_experiment-1-0.arrow
the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you.
OK, it definitely leads to a race condition in how it's used right now. Here is how you can reproduce it - by injecting a random sleep time different for each process before the locks are acquired.
```
--- a/src/datasets/metric.py
+++ b/src/datasets/metric.py
@@ -348,6 +348,16 @@ class Metric(MetricInfoMixin):
elif self.process_id == 0:
# Let's acquire a lock on each node files to be sure they are finished writing
+
+ import time
+ import random
+ import os
+ pid = os.getpid()
+ random.seed(pid)
+ secs = random.randint(1, 15)
+ time.sleep(secs)
+ print(f"sleeping {secs}")
+
file_paths, filelocks = self._get_all_cache_files()
# Read the predictions and references
@@ -385,7 +395,10 @@ class Metric(MetricInfoMixin):
if predictions is not None:
self.add_batch(predictions=predictions, references=references)
+ print("FINALIZE START")
+
self._finalize()
+ print("FINALIZE END")
self.cache_file_name = None
self.filelock = None
```
then run with 2 procs: `python -m torch.distributed.launch --nproc_per_node=2`
```
export BS=16; rm -r output_dir; PYTHONPATH=src USE_TF=0 CUDA_VISIBLE_DEVICES=0,1 python -m torch.distributed.launch --nproc_per_node=2 examples/seq2seq/run_seq2seq.py --model_name_or_path t5-small --output_dir output_dir --adam_eps 1e-06 --do_eval --do_train --do_predict --evaluation_strategy=steps --label_smoothing 0.1 --learning_rate 3e-5 --logging_first_step --logging_steps 1000 --max_source_length 128 --max_target_length 128 --num_train_epochs 1 --overwrite_output_dir --per_device_eval_batch_size $BS --per_device_train_batch_size $BS --predict_with_generate --eval_steps 25000 --sortish_sampler --task translation_en_to_ro --val_max_target_length 128 --warmup_steps 500 --max_train_samples 10 --max_val_samples 10 --max_test_samples 10 --dataset_name wmt16 --dataset_config ro-en --source_prefix "translate English to Romanian: "
```
```
***** Running Evaluation *****
Num examples = 10
Batch size = 16
0%| | 0/1 [00:00<?, ?it/s]FINALIZE START
FINALIZE START
sleeping 11
FINALIZE END
100%|██████████████████████████████████████████████████████████████████████████████████████████████████████████████████████████████| 1/1 [00:11<00:00, 11.06s/it]
sleeping 11
Traceback (most recent call last):
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/metric.py", line 368, in _finalize
self.data = Dataset(**reader.read_files([{"filename": f} for f in file_paths]))
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/arrow_reader.py", line 236, in read_files
pa_table = self._read_files(files, in_memory=in_memory)
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/arrow_reader.py", line 171, in _read_files
pa_table: pa.Table = self._get_dataset_from_filename(f_dict, in_memory=in_memory)
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/arrow_reader.py", line 302, in _get_dataset_from_filename
pa_table = ArrowReader.read_table(filename, in_memory=in_memory)
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/arrow_reader.py", line 322, in read_table
stream = stream_from(filename)
File "pyarrow/io.pxi", line 782, in pyarrow.lib.memory_map
File "pyarrow/io.pxi", line 743, in pyarrow.lib.MemoryMappedFile._open
File "pyarrow/error.pxi", line 122, in pyarrow.lib.pyarrow_internal_check_status
File "pyarrow/error.pxi", line 97, in pyarrow.lib.check_status
FileNotFoundError: [Errno 2] Failed to open local file '/home/stas/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow'. Detail: [errno 2] No such file or directory
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "examples/seq2seq/run_seq2seq.py", line 645, in <module>
main()
File "examples/seq2seq/run_seq2seq.py", line 601, in main
metrics = trainer.evaluate(
File "/mnt/nvme1/code/huggingface/transformers-mp-pp/src/transformers/trainer_seq2seq.py", line 74, in evaluate
return super().evaluate(eval_dataset, ignore_keys=ignore_keys, metric_key_prefix=metric_key_prefix)
File "/mnt/nvme1/code/huggingface/transformers-mp-pp/src/transformers/trainer.py", line 1703, in evaluate
output = self.prediction_loop(
File "/mnt/nvme1/code/huggingface/transformers-mp-pp/src/transformers/trainer.py", line 1876, in prediction_loop
metrics = self.compute_metrics(EvalPrediction(predictions=preds, label_ids=label_ids))
File "examples/seq2seq/run_seq2seq.py", line 556, in compute_metrics
result = metric.compute(predictions=decoded_preds, references=decoded_labels)
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/metric.py", line 402, in compute
self._finalize()
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/metric.py", line 370, in _finalize
raise ValueError(
ValueError: Error in finalize: another metric instance is already using the local cache file. Please specify an experiment_id to avoid colision between distributed metric instances.
``` | [
-0.0844155252,
-0.0657196268,
0.0652178898,
0.2722368836,
-0.0870860815,
-0.0326647907,
0.1643526852,
0.2697965801,
0.3311938345,
0.0832056925,
0.0982609913,
0.1941851974,
-0.3580646515,
-0.0889754444,
-0.0156052159,
0.0498046838,
0.0008351952,
-0.1240272,
-0.4149838686,
-0.1004128158,
-0.349976629,
0.5602377057,
-0.1571678221,
0.0035177856,
-0.4620629847,
0.1669692695,
-0.1928112507,
0.2385936528,
-0.105525054,
-0.569846034,
0.2600385845,
0.3488969505,
-0.017399272,
0.5673114657,
-0.0001218659,
-0.1565489322,
0.2898033261,
-0.1259327233,
-0.0928893164,
-0.1496434659,
0.0516418628,
-0.3085163236,
0.4341695011,
-0.1971870214,
-0.0820826963,
-0.0098866438,
0.0758306757,
-0.4951308966,
0.4286835194,
0.0644458607,
0.0891191065,
0.46892941,
-0.0374807417,
-0.1865042448,
-0.0093003381,
-0.1247956455,
0.0236410964,
0.3594596386,
-0.0119027784,
0.005028117,
-0.2551396489,
0.2341877967,
0.235095039,
-0.0686762184,
0.363065213,
0.0002418762,
-0.023256788,
-0.1683157533,
-0.1944672763,
0.3529825509,
0.1351853609,
-0.1981306672,
-0.2590883076,
0.0288157016,
-0.1893538386,
-0.4624346197,
0.1429928839,
-0.1314377785,
0.0301053207,
0.0880872533,
0.0458046272,
0.0006789182,
0.0064209024,
0.0480428152,
-0.0750624686,
0.0268835258,
-0.1102875844,
0.0966648683,
-0.0770764574,
0.2674497962,
-0.3081118762,
0.2120123655,
-0.0161629133,
0.1204220429,
-0.4356836677,
0.0894818306,
0.2162852287,
0.0534019284,
0.0726227015,
0.4313856959,
-0.0319298692,
0.1022295505,
0.3979120851,
0.1139201671,
-0.0671370551,
0.4132385552,
0.2391604483,
-0.0104751568,
0.1874963343,
0.2739360929,
-0.0854606107,
-0.2305918634,
0.2011026889,
-0.2459426224,
0.3607816994,
0.0959144831,
0.1355986446,
-0.3899982274,
0.0805315003,
0.30113855,
-0.0750803426,
-0.1568285376,
0.1580273211,
0.3855431974,
0.0852395296,
-0.0463727564,
0.3243297935,
0.3055721819,
-0.2333541662,
0.0019364143,
-0.4296100736,
-0.2353733629,
-0.1194879562,
0.3071151376,
0.05910822,
-0.0130564421,
0.6048253179,
-0.1873203814,
0.3196307421,
-0.0907877982,
0.3079951108,
0.1744772196,
0.0746119022,
0.2866276801,
-0.0819512829,
0.185196206,
0.3427526355,
0.1244144663,
-0.2457848489,
-0.2860184014,
-0.0978618711,
-0.4152009189,
0.1885588616,
0.0005309858,
-0.5068398118,
0.2394953966,
0.4041300118,
0.2187767923,
-0.0533615388,
-0.1476348639,
0.2692392468,
-0.0410024598,
-0.1580676436,
-0.0170981344,
0.4377285838,
0.8433100581,
-0.2321902066,
-0.4020534754,
0.1269696504,
-0.0551429987,
0.0210925415,
0.2261463106,
-0.0151569918,
0.1313894391,
-0.2769351304,
0.1363206208,
0.3145764172,
-0.3304305077,
-0.5092276931,
-0.0667771995,
-0.4374722838,
-0.0340689905,
0.1925943643,
0.109986268,
0.0847025216,
0.1234844178,
0.1166358516,
-0.2643077374,
0.2656231523,
-0.3458692133,
-0.3824669719,
-0.2897081673,
-0.037065845,
0.0175564028,
0.3021222353,
0.0015972788,
-0.0653042123,
0.0374570228,
0.1378031373,
0.1916086674,
0.1767646521,
0.2403719872,
0.235022679,
0.0061784578,
0.2478130162,
0.1133007929,
-0.2364608198,
0.3414191604,
-0.382301271,
0.0915210471,
0.184273541,
0.0344476178,
-0.1589945555,
-0.1162983924,
-0.1790623218,
-0.4125054777,
0.0269139148,
-0.1299065948,
0.1790641993,
0.1748149693,
-0.1957101822,
0.1845991313,
-0.0311742313,
0.2445206344,
-0.4866952598,
0.080915682,
-0.0441645309,
-0.360732168,
-0.0655889437,
0.0390102565,
0.0376203097,
0.0411499925,
-0.1389890015,
0.368803829,
-0.1038147882,
0.2514377832,
0.2193903178,
0.2252398878,
-0.0583164282,
-0.330986768,
-0.1056120545,
0.1239272803,
0.0039936979,
-0.0451716259,
-0.1759319901,
0.3884066045,
0.0900152251,
0.1461753696,
-0.3499468863,
0.2182744592,
-0.0972634405,
-0.1663036197,
-0.555668056,
-0.0380488783,
0.4728537798,
-0.2647348344,
0.2770220339,
-0.163131699,
0.0137210656,
0.0223104525,
0.2333168089,
0.1545522958,
0.1547574848,
0.0433733724,
-0.2333092391,
-0.0644803867,
0.0418287888,
-0.1615595222,
0.4600604475,
0.1955894679,
0.2431959063,
0.1327683181,
0.0874554813,
-0.2459524423,
0.0757679641,
0.0206986275,
-0.1691023558,
0.1593049467,
0.1514808387,
0.1435251385,
-0.3288491368,
-0.138001591,
-0.2743545473,
0.0329184383,
-0.3341396749,
0.1020792201,
-0.134984538,
0.4001254141,
0.1546523869,
0.064531289,
-0.1272898763,
-0.2716539502,
0.1869914234,
-0.1014484912,
0.0305640735,
0.2809855938,
-0.2944431305,
0.530046761,
0.110001877,
-0.1811982989,
-0.3561825156,
-0.2111855447,
-0.0076868478,
-0.1622641981,
-0.1794361919,
0.193083033,
0.0939461291,
-0.1835871637,
-0.0286588706,
-0.0898883864,
-0.3543088138,
0.0646217093,
0.0579993315,
0.6808117628,
0.2862640023,
0.0012739364,
-0.2250636071,
0.0685056522,
0.4061903358,
-0.4061915278,
-0.31842044,
0.0798086897,
-0.0271715689,
0.136747241,
-0.3171878457,
-0.2778087854,
0.1093107015,
-0.3724223375,
0.6279047728,
0.0351588801,
-0.0284732431,
-0.1151443049,
-0.006565142,
0.2452504188,
-0.2081533819,
0.2181215733,
-0.3708447516,
-0.6790496111,
0.3761589229,
-0.0701829568,
-0.2920276225,
-0.1314426363,
0.0402267464,
0.3392745256,
-0.0791690499,
-0.59269768,
-0.522321403,
-0.0324284844,
0.3241591752,
0.0222350899,
-0.0101589104,
0.5464062095,
-0.0936020687,
-0.0244744886,
-0.0935699567,
-0.1157883406,
0.6498821378,
-0.160006538,
0.1253626794,
-0.0418157727,
0.5212671757,
0.1402979493,
0.9083521366,
0.5984094143,
0.1078084409,
0.2427326292,
0.0859566852,
0.37660864,
-0.1323871315,
-0.1446453482,
0.3193693757,
0.1811807305,
-0.1200468764,
0.1948684752,
0.0696765184,
0.1736306995,
-0.0670454949,
0.0006564169,
-0.2872374952,
-0.3173186481,
-0.0860207528,
-0.0220396183,
0.1946453005,
0.0260200817,
0.0464019515,
-0.1714588106,
0.021022886,
0.4557758868,
0.4667411149,
0.2445891052,
0.1518063992,
-0.2346047759,
0.150271818,
-0.6847792268,
0.2808539867,
-0.4843690097,
0.0926618278,
-0.2083666474,
-0.2226662934,
0.1251804233,
-0.1038857549,
0.561988771,
0.1111256182,
-0.0981004238,
0.038946785,
-0.2003228962,
-0.4362588525,
-0.0758718029,
-0.1520859599,
-0.0548394918,
0.3091388941,
0.4101701677,
-0.4372712374,
-0.2195555866,
-0.1674848497,
-0.1153562292,
-0.2638754547,
-0.3710044324,
-0.3840123415,
-0.0693475306,
-0.1728612185,
0.2685464323,
-0.149405092,
-0.0337942056,
-0.0002120398,
-0.0176425781,
-0.1778661013,
-0.1178580746,
0.0093378611,
0.1683957577,
0.3239504397,
-0.3101280034,
0.261333257,
0.5088199973,
-0.2863686383,
0.0070696333,
0.3720150888,
0.112595059,
0.0114654507,
0.3537887633,
-0.0389567539,
0.1451953202,
0.6060225964,
-0.2288957387,
0.1373127252,
0.0775090307,
0.3824472427,
-0.1733541489,
-0.0328599289,
0.3560642898,
-0.0646376535,
0.3657296896,
0.194378987,
0.2134577334,
0.2551452816,
-0.2164089382,
0.3798716664,
0.0024196121,
-0.125467658,
0.289678365,
0.0662680045,
0.9412876368,
0.1287700683,
0.1316241622,
0.3074278831,
0.030038856,
0.3580704629,
0.1575553566,
0.0769379139,
-0.215500325,
-0.2878908813,
0.0281257872,
-0.1426006705,
0.0565015003,
-0.2786585093,
-0.1992246062,
0.3468228877,
-0.3472925723,
-0.1368991435,
-0.2096108496,
-0.1000207886,
-0.2952027023,
-0.1441334933,
0.1108290777,
0.0379241519,
0.0561158843,
0.5053173304,
-0.1962721646,
-0.1456763893,
-0.3237815797,
-0.2361002713,
-0.0989165753,
-0.0192421544,
-0.212822929,
0.0031839847,
0.2255653739,
-0.0905268341,
-0.1694489121,
0.2623282075,
0.4520953894,
0.1580690593,
-0.2806239724,
0.0806226656,
0.1305503696,
0.05935321,
0.1180901527,
-0.0961391404,
0.2394873947,
-0.1169214472,
-0.0971451774,
0.0897524133,
-0.2411243618,
0.0596833415,
0.0625080913,
-0.1247527972,
-0.0613276213,
-0.0006806592,
0.018707877,
0.174497813,
-0.0133294072,
-0.2417228222,
0.0776690766,
0.3355979621,
-0.1957993954,
0.0846764222,
0.1114367992,
-0.2621312141,
-0.1653985679,
0.5599820614,
-0.1930414289,
-0.0438051373,
0.4942459464,
0.0620816424,
-0.0214790609,
-0.0895951688,
0.0665493309,
0.4614337385,
-0.7272005081,
0.1340643317,
-0.0469328761,
-0.0091642961,
0.1100815088,
0.2127387226,
0.3792603016,
-0.1826426685,
-0.088615723,
-0.17027466,
-0.3497693837,
0.2320085317,
-0.3465443254,
0.1859751046,
-0.4120007455,
0.0863511935,
-0.2553624809,
0.2320758849,
-0.2822773159,
0.1403428018,
-0.3109701872,
-0.1574934721,
-0.0858476609,
-0.1043038145,
0.3523843586,
-0.0494925231,
-0.0698429197,
0.1268847138,
-0.4691964388,
-0.101558879,
-0.2797078788,
0.1388323754,
-0.1304949075,
0.0779668465,
-0.0051859305,
0.2057711333,
-0.0304873921,
-0.0940724611,
0.0187170561,
0.1957747787,
0.0266361088,
0.0420337282,
0.2091131657,
0.1399056315,
-0.1949336529,
0.1630111635,
0.0852944925,
-0.2907068431,
-0.1156036407,
-0.0107713947,
-0.298253715,
-0.0722030401,
0.167250514,
0.1254225522,
-0.1080610603,
0.1442046613,
0.1264521629,
-0.0076735131,
-0.300779283,
-0.0219409354,
0.4440123439,
0.1493701488,
-0.1383355111,
-0.1004374847,
-0.1468461007,
0.0966835171,
-0.0956379697,
0.2848829329,
-0.0055988696,
0.35734424,
0.1428940892,
0.2579459846,
0.1733796299,
0.0006110123,
0.0838577524,
0.287468344,
0.033803232,
-0.1745363027,
0.290810138,
-0.212521717,
0.1121103913,
0.3444501758,
0.6617324948,
0.0436841995,
0.1996882558,
-0.1315071434,
-0.0667859092,
0.2362421602,
-0.1090037972,
0.3268864453,
-0.2201715112,
-0.1009960771,
-0.1156373024,
0.070099704,
-0.1066151112,
-0.133678481,
-0.3784922957,
0.6685899496,
-0.3151123822,
-0.2031566799,
-0.184766233,
0.2426724434,
-0.135775432,
-0.2470302284,
-0.1185155436,
-0.1595747024,
-0.0961112007,
-0.1728563309,
0.2104007453,
0.0722269341,
0.1171075404,
0.0522645675,
0.0329215527,
-0.2532563508,
-0.4568679631,
0.2618016601,
0.2318311185,
-0.2234421521,
0.0250681154,
0.2808710337,
-0.3499057889,
0.1182909459,
0.6035223007,
0.2323000431,
-0.0582312085,
-0.0834592283,
0.1073073447,
0.3267664015,
0.0169564467,
-0.0994199738,
0.1862422675,
0.3138246834,
-0.5439273715,
0.0629943386,
0.0679737478,
-0.1795364767,
0.3942137063,
-0.2318454385,
0.3350016177,
-0.2536055446,
0.4101297259,
-0.2309689224,
0.1492196172,
0.0442677513,
-0.1309384257,
-0.6219240427,
0.2565178573,
-0.0546290167,
-0.0505177304,
0.0629609451,
-0.2929469943,
0.0455986299,
-0.1245392188,
0.2503247559,
0.3252750337,
0.0252176467,
-0.3522541225,
-0.1959873289,
-0.5977159739,
0.2946240902,
0.1455522329,
0.2486118525,
-0.0141240619,
0.3637275994,
-0.0974160135,
0.0827246159,
0.1792982966,
-0.0695220008,
-0.1520160437,
0.0536885485,
-0.5792642236,
0.1903706789,
-0.1522356123,
-0.1525033414,
0.1285754889,
0.1937537193,
0.0038235404,
-0.2565793991,
0.0009939986,
0.2496806681,
-0.2043686509,
0.0098011512,
0.2409564257,
0.4613413811,
0.1374108642,
0.0890942514,
0.0119942008,
-0.0801087543,
-0.2506814897,
-0.1601438075,
-0.1050342321,
-0.1749793291,
-0.3328831792,
0.4853778183,
-0.3128303587,
-0.5159757137,
-0.2895018458,
0.2952622175,
0.0879573748,
-0.3334015012,
-0.2367615998,
0.3818423748,
-0.1371768713,
0.0356157459,
0.2386958152,
0.3839944601,
0.0910455287,
0.3809012175,
-0.3290144503,
-0.1753952205,
0.7739756703,
-0.5265746117,
0.2481271774,
-0.2379906923,
0.3535303473,
0.4005734921,
-0.3686008155,
-0.2991080284,
-0.0865049958,
0.1388595551,
0.0136335799,
-0.1585734487,
0.1545863599,
-0.3073342443,
-0.0271866024,
-0.0483863242,
0.1458696276,
0.1749284714,
-0.0491092652,
-0.0030155401,
-0.1477311999
]
|
https://github.com/huggingface/datasets/issues/1942 | [experiment] missing default_experiment-1-0.arrow | I tried to adjust `run_seq2seq.py` and trainer to use the suggested dist env:
```
import torch.distributed as dist
metric = load_metric(metric_name, num_process=dist.get_world_size(), process_id=dist.get_rank())
```
and in `trainer.py` added to call just for rank 0:
```
if self.is_world_process_zero() and self.compute_metrics is not None and preds is not None and label_ids is not None:
metrics = self.compute_metrics(EvalPrediction(predictions=preds, label_ids=label_ids))
```
and then the process hangs in a deadlock.
Here is the tb:
```
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/utils/filelock.py", line 275 in acquire
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/metric.py", line 306 in _check_all_processes_locks
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/metric.py", line 501 in _init_writer
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/metric.py", line 440 in add_batch
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/metric.py", line 397 in compute
File "examples/seq2seq/run_seq2seq.py", line 558 in compute_metrics
File "/mnt/nvme1/code/huggingface/transformers-mp-pp/src/transformers/trainer.py", line 1876 in prediction_loop
File "/mnt/nvme1/code/huggingface/transformers-mp-pp/src/transformers/trainer.py", line 1703 in evaluate
File "/mnt/nvme1/code/huggingface/transformers-mp-pp/src/transformers/trainer_seq2seq.py", line 74 in evaluate
File "examples/seq2seq/run_seq2seq.py", line 603 in main
File "examples/seq2seq/run_seq2seq.py", line 651 in <module>
```
But this sounds right, since in the above diff I set up a distributed metric and only called one process - so it's blocking on waiting for other processes to do the same.
So one working solution is to leave:
```
metric = load_metric(metric_name)
```
alone, and only call `compute_metrics` from rank 0
```
if self.is_world_process_zero() and self.compute_metrics is not None and preds is not None and label_ids is not None:
metrics = self.compute_metrics(EvalPrediction(predictions=preds, label_ids=label_ids))
```
so we now no longer use the distributed env as far as `datasets` is concerned, it's just a single process.
Are there any repercussions/side-effects to this proposed change in Trainer? If it always gathers all inputs on rank 0 then this is how it should have been done in first place - i.e. only run for rank 0. It appears that currently it was re-calculating the metrics on all processes on the same data just to throw the results away other than for rank 0. Unless I missed something.
| the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you. | 302 | [experiment] missing default_experiment-1-0.arrow
the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you.
I tried to adjust `run_seq2seq.py` and trainer to use the suggested dist env:
```
import torch.distributed as dist
metric = load_metric(metric_name, num_process=dist.get_world_size(), process_id=dist.get_rank())
```
and in `trainer.py` added to call just for rank 0:
```
if self.is_world_process_zero() and self.compute_metrics is not None and preds is not None and label_ids is not None:
metrics = self.compute_metrics(EvalPrediction(predictions=preds, label_ids=label_ids))
```
and then the process hangs in a deadlock.
Here is the tb:
```
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/utils/filelock.py", line 275 in acquire
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/metric.py", line 306 in _check_all_processes_locks
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/metric.py", line 501 in _init_writer
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/metric.py", line 440 in add_batch
File "/mnt/nvme1/code/huggingface/datasets-master/src/datasets/metric.py", line 397 in compute
File "examples/seq2seq/run_seq2seq.py", line 558 in compute_metrics
File "/mnt/nvme1/code/huggingface/transformers-mp-pp/src/transformers/trainer.py", line 1876 in prediction_loop
File "/mnt/nvme1/code/huggingface/transformers-mp-pp/src/transformers/trainer.py", line 1703 in evaluate
File "/mnt/nvme1/code/huggingface/transformers-mp-pp/src/transformers/trainer_seq2seq.py", line 74 in evaluate
File "examples/seq2seq/run_seq2seq.py", line 603 in main
File "examples/seq2seq/run_seq2seq.py", line 651 in <module>
```
But this sounds right, since in the above diff I set up a distributed metric and only called one process - so it's blocking on waiting for other processes to do the same.
So one working solution is to leave:
```
metric = load_metric(metric_name)
```
alone, and only call `compute_metrics` from rank 0
```
if self.is_world_process_zero() and self.compute_metrics is not None and preds is not None and label_ids is not None:
metrics = self.compute_metrics(EvalPrediction(predictions=preds, label_ids=label_ids))
```
so we now no longer use the distributed env as far as `datasets` is concerned, it's just a single process.
Are there any repercussions/side-effects to this proposed change in Trainer? If it always gathers all inputs on rank 0 then this is how it should have been done in first place - i.e. only run for rank 0. It appears that currently it was re-calculating the metrics on all processes on the same data just to throw the results away other than for rank 0. Unless I missed something.
| [
0.0881398842,
-0.1092866957,
0.1158301532,
0.2856830657,
0.0845730975,
0.0806555673,
0.2349970043,
0.2377155423,
0.2347910851,
0.2356439382,
0.0788628757,
0.1667125374,
-0.3739603162,
-0.2242654413,
0.0668829158,
0.0503918231,
-0.0005046276,
-0.1439885944,
-0.22103028,
-0.1951078475,
-0.2040554136,
0.5319713354,
-0.0888305828,
-0.0502558351,
-0.5913399458,
0.2881838977,
-0.1651009917,
0.1823535711,
-0.0684027225,
-0.6171510816,
0.3700886667,
0.1490113735,
0.0829517245,
0.5546144247,
-0.0001318881,
-0.1994536668,
0.2629816532,
-0.2211296111,
-0.153406173,
-0.2687930763,
0.1527821869,
-0.2575646341,
0.57150352,
-0.1630464792,
-0.1416181922,
-0.0879457593,
0.0900790766,
-0.4483619332,
0.4259666204,
0.1269227266,
-0.0067990683,
0.3528018892,
-0.0033906889,
-0.2833949029,
0.0977117941,
-0.1208253503,
0.0339140445,
0.3804102838,
-0.0241649505,
0.0620027669,
-0.3279882967,
0.206839174,
0.2323786318,
-0.0643237904,
0.3330112398,
-0.0780321434,
-0.1014688686,
-0.1370964199,
-0.0787226632,
0.3096214831,
0.2080832571,
-0.1693376452,
-0.2303848565,
0.0603588335,
0.0139126126,
-0.3432676196,
0.1953569353,
-0.0388792343,
-0.0130723557,
0.0416940488,
0.0024042998,
-0.1951524764,
0.0043456121,
0.0744461343,
-0.1773835719,
0.1295823753,
-0.0661411583,
0.0334934331,
0.0162891932,
0.2591890395,
-0.1607431471,
0.3018883765,
0.0713187605,
0.1371361315,
-0.3619205654,
0.1703316867,
0.2666285038,
0.0578646846,
-0.0056572277,
0.3195265532,
-0.036185421,
0.0114495782,
0.4184843004,
0.0381958447,
-0.1389735937,
0.3627062142,
0.2786944211,
0.1029437557,
0.1162616909,
0.1878528148,
-0.0855456591,
-0.2297567725,
0.0198363494,
-0.1975077391,
0.3149442971,
0.2442041337,
-0.0334213749,
-0.420573324,
0.1195660904,
0.2952426374,
-0.1290900856,
-0.0933245867,
0.16934295,
0.3671612144,
0.0882648006,
0.0787011608,
0.335329473,
0.24716793,
-0.1991894394,
-0.0173157752,
-0.4135640562,
-0.0986427292,
-0.1242090166,
0.2724474967,
0.2196633816,
-0.162900731,
0.6351845264,
-0.1787697971,
0.4696892798,
-0.0300205443,
0.1313091815,
0.1857782006,
0.1316874325,
0.2409881204,
-0.0306471549,
0.2101944089,
0.3361890018,
0.3006997406,
-0.1925241649,
-0.1458502412,
-0.1428040862,
-0.5472250581,
0.1147266105,
-0.0964164138,
-0.4572975338,
0.2726357877,
0.3576212525,
0.111712195,
-0.1076126695,
-0.1904318333,
0.1932598054,
-0.0918902084,
-0.0997681171,
-0.0660915896,
0.5843694806,
0.7293351889,
-0.2147575468,
-0.4012364447,
0.0988394469,
0.0325715095,
-0.1022928879,
0.152945444,
-0.0788160488,
0.1933200061,
-0.3543679714,
-0.0127081042,
0.3378304839,
-0.4275303185,
-0.5466862321,
-0.1503926069,
-0.3531806767,
-0.1286652982,
0.215179652,
0.0550853163,
0.1297100186,
0.1625277996,
0.1186123639,
-0.1818706244,
0.1395099163,
-0.3199318945,
-0.2878007591,
-0.1782019436,
0.0546485409,
0.004848049,
0.3287586272,
-0.0217357744,
-0.0645742789,
0.1194038987,
0.1203887016,
0.1731574535,
0.142739892,
0.3467542529,
0.1570515782,
0.0381507128,
0.2471780628,
0.136305213,
-0.3056513369,
0.3053780496,
-0.3536008298,
0.0743500441,
0.0327290781,
0.0219309032,
-0.1138971746,
-0.2049703151,
-0.1658815295,
-0.4732398689,
-0.1058144197,
-0.0443965159,
0.1530438364,
0.1016913056,
-0.1681245267,
0.1105812639,
-0.0655054897,
0.271903187,
-0.4879297316,
0.0612641424,
-0.0638747439,
-0.3398910165,
-0.0635266826,
0.1616178304,
0.0212045852,
0.0049572308,
-0.1978688985,
0.3824713528,
-0.1466091424,
0.216760844,
0.252396524,
0.1297357231,
-0.0266659167,
-0.5362625718,
-0.0249715522,
0.1701556891,
-0.0606265925,
0.0106332293,
-0.2244264185,
0.3717920482,
0.1169157103,
0.152842477,
-0.3292897046,
0.2574850023,
-0.1934432983,
-0.0311922859,
-0.4751604497,
-0.0524112545,
0.4612511694,
-0.1249243543,
0.3616277575,
-0.0769222602,
0.0478942581,
-0.0536689647,
0.1575226784,
0.1602756381,
0.0998462215,
0.0825530589,
-0.1949328631,
-0.0521236435,
0.0845896304,
-0.2958513796,
0.4181764424,
0.185945645,
0.2945017219,
0.0671906844,
0.1383648664,
-0.1661347449,
0.0406896621,
-0.0327740759,
-0.1795001477,
0.2156122029,
0.2768220901,
0.1916082054,
-0.2365641892,
-0.0302204303,
-0.2584660947,
0.0539822169,
-0.3396648169,
0.0545163713,
-0.2114025354,
0.5269722939,
0.1609438211,
-0.0017836258,
-0.3060410917,
-0.2587699592,
0.198584348,
-0.1509749591,
0.107367456,
0.2856148779,
-0.1824018061,
0.3663707674,
0.1217023209,
-0.1664307714,
-0.2195236981,
-0.2145322561,
-0.0526188351,
-0.261505276,
-0.2276444137,
-0.0176191982,
0.1051157713,
-0.1812951267,
-0.0146132726,
-0.0838482231,
-0.3236864805,
0.0789874196,
0.0525832139,
0.5157328248,
0.3757799268,
0.0781344473,
-0.3018796444,
0.1728726327,
0.4036900401,
-0.4335688353,
-0.2911327183,
0.0549029261,
0.0282637216,
0.2603510618,
-0.2404190898,
-0.3637054861,
0.0195959918,
-0.3122051358,
0.4976966083,
0.0147305895,
-0.06709674,
-0.2401186079,
0.015915079,
0.2207767367,
-0.1401596367,
0.3164169788,
-0.2179738581,
-0.7374212146,
0.4974016547,
-0.0844748989,
-0.2427445948,
-0.0719337389,
0.0304231737,
0.3310315907,
0.0592322834,
-0.6748086214,
-0.377584666,
0.0442799479,
0.2911629975,
-0.1235283539,
0.1387413442,
0.5976378322,
-0.0984462574,
0.0906326771,
-0.0848378316,
-0.1646195352,
0.5146776438,
-0.2370685786,
0.2622248232,
-0.0529931262,
0.3953696191,
0.2051372379,
1.0660389662,
0.4365730286,
0.1375795156,
0.2404037863,
0.0790977776,
0.3862740695,
-0.1463312805,
-0.1985455751,
0.292593807,
0.2944894731,
-0.0125391483,
0.1614472121,
0.104041256,
0.2343330532,
-0.1193144768,
-0.0580517054,
-0.2382647395,
-0.2966469228,
0.0124177616,
-0.246753037,
0.2885680199,
-0.076827623,
0.1307218969,
-0.2336003333,
-0.030970294,
0.4748419523,
0.3607446849,
0.3400710821,
0.2659282684,
0.0138888294,
0.0185920671,
-0.5698677897,
0.2131745964,
-0.3507133424,
0.0432461314,
-0.1114051268,
-0.2091224939,
0.1995762289,
-0.0166741535,
0.6270529032,
0.259426415,
-0.132365644,
-0.0344229043,
-0.3146465719,
-0.4235263169,
-0.0951625481,
0.0202625748,
-0.0807243809,
0.261980623,
0.2443540245,
-0.4367319047,
-0.2846648395,
-0.0777887106,
-0.0575863868,
-0.2513467371,
-0.3316437006,
-0.2910424173,
0.0722149685,
-0.2103280425,
0.1533395499,
-0.1221065745,
0.015193332,
-0.0006407864,
0.0610139295,
-0.1972709,
-0.0585681461,
0.005117245,
0.0079775648,
0.3492985666,
-0.3426239491,
0.1846986115,
0.5938442349,
0.0462312587,
0.0694636405,
0.3731180727,
0.0241028555,
-0.0076119588,
0.3295190334,
-0.1096443087,
0.1984229237,
0.6668336391,
-0.2204793245,
0.0092968252,
-0.1022755355,
0.2657600343,
-0.0840349719,
-0.024089152,
0.2751719654,
-0.1857891232,
0.3366444707,
0.1338972747,
0.1865535825,
0.3631663024,
-0.0754489675,
0.3928916156,
0.0175172016,
-0.1314399838,
0.169727385,
0.0900111571,
1.0596711636,
0.1733085811,
0.2880569696,
0.0718042254,
0.1134337634,
0.286826849,
0.0770511925,
0.0670694783,
-0.2336567044,
-0.2930977046,
-0.0237973705,
-0.2060995102,
-0.0268656537,
-0.2503249347,
-0.0878033116,
0.4228730798,
-0.3943591416,
-0.0798697695,
-0.209823817,
-0.1599922329,
-0.2157910466,
-0.1572387964,
0.1809961051,
-0.0825242475,
0.0263454858,
0.5747039318,
-0.215761438,
-0.1310920715,
-0.3793374002,
-0.3467715681,
-0.1881609708,
-0.0683696121,
-0.3320907056,
0.0041431524,
0.332113415,
-0.3493466973,
-0.1050178334,
0.4051664472,
0.4119633734,
0.1500436813,
-0.315889895,
0.0133934366,
0.1522024721,
0.0163301565,
0.066248484,
0.0037691968,
0.214513436,
-0.0231949147,
-0.0367357209,
0.1018837094,
-0.2719016969,
0.0721195415,
0.0464074984,
-0.1299455911,
0.1035779193,
0.0079812277,
-0.0395117253,
0.0416477546,
0.0088515729,
-0.224610433,
0.0092117498,
0.3180169761,
-0.3020226061,
-0.1260165423,
0.1327935606,
-0.2384745181,
-0.0902607441,
0.7388561368,
-0.1895922869,
-0.0833806768,
0.5086651444,
0.1132062003,
-0.0950123742,
-0.0279414859,
0.0534408353,
0.5871479511,
-0.7964068055,
0.0375652164,
-0.0280947722,
-0.0733697861,
0.0531306043,
0.1573777795,
0.4190031588,
-0.1953765452,
-0.0511976294,
-0.1429458112,
-0.5170994997,
0.1747763008,
-0.4015874267,
0.2136111259,
-0.4579899013,
0.1037901565,
-0.120552443,
0.1368552148,
-0.1713809967,
0.2145241946,
-0.1843113005,
-0.0760748982,
-0.0796442032,
-0.0408800766,
0.2494169921,
-0.0830161497,
-0.1323853582,
0.1427086145,
-0.2992048562,
-0.0110410973,
-0.2597684562,
0.2003601044,
-0.1354682595,
0.1764082462,
-0.0286941249,
0.1227869093,
-0.0617898516,
-0.1407513618,
0.0586237274,
0.2382396907,
0.1416049004,
0.1680944413,
0.1831720173,
0.2058738619,
-0.1489623934,
0.1856941432,
0.1167299449,
-0.1866596043,
-0.1223105863,
-0.0412708484,
-0.31142506,
-0.0305347648,
0.0741105825,
0.1024202928,
-0.1661488563,
0.2045537829,
0.0971293598,
0.0333638601,
-0.2555372119,
0.0163984597,
0.3647415042,
0.0902381092,
-0.2043002993,
0.0552670509,
-0.0069349171,
0.0217384994,
-0.1006233618,
0.2891531587,
0.0024504585,
0.3737569749,
-0.0071062655,
0.2339391708,
0.1555731595,
-0.0368142501,
0.0814677998,
0.2663897872,
-0.0957862958,
-0.1646015495,
0.3494299352,
-0.0394397341,
0.1696408391,
0.2915655077,
0.5672033429,
0.1148113906,
0.1399040967,
-0.0718221292,
-0.093902126,
0.3427059948,
-0.1072871014,
0.2498675138,
-0.1057757735,
-0.2143326849,
-0.2015597075,
-0.010069645,
0.1364569068,
-0.2415561974,
-0.4650443792,
0.7891872525,
-0.2494345158,
-0.2857099771,
-0.1221795604,
0.2552677393,
-0.1764251292,
-0.1262227446,
-0.0582017116,
-0.1612550616,
-0.1629289389,
-0.0820107311,
0.2177404314,
-0.0160786603,
0.1115117595,
0.2160970271,
-0.0037840942,
-0.3101453185,
-0.4137938023,
0.2377392352,
0.221997574,
-0.1553963125,
0.0707511455,
0.1623301953,
-0.2955520749,
0.1464755237,
0.5265612602,
0.1610319167,
0.0471728109,
-0.1688612998,
0.0138864918,
0.3292219639,
-0.0358101241,
-0.0913412347,
0.1298604757,
0.212679401,
-0.4804818928,
-0.0339948162,
-0.0184380636,
-0.104944624,
0.3532825708,
-0.2245301306,
0.2486274093,
-0.1422256827,
0.5203974843,
-0.1859231591,
0.0720939711,
0.046000883,
-0.1357634962,
-0.6475101709,
0.2183961719,
-0.108709015,
0.074786,
0.0200168099,
-0.2638199031,
-0.0101508992,
-0.1398232728,
0.1454686224,
0.4622712433,
0.0498205163,
-0.4014341831,
-0.2744064033,
-0.5686837435,
0.3184739649,
0.2554923892,
0.2237135768,
-0.0390213951,
0.27680251,
-0.0909411535,
0.0218144096,
0.1413946301,
-0.0164729692,
0.1134109646,
0.1315147132,
-0.5518437624,
0.1067016348,
-0.1301145554,
-0.0802232549,
0.0674611107,
0.3303527534,
-0.1294990629,
-0.1872289032,
-0.0767546967,
0.1827466488,
-0.2880125344,
-0.0719872937,
0.3570815325,
0.3660235703,
-0.0069944141,
0.1416060627,
0.0799733996,
-0.1299113631,
-0.2005254626,
-0.0048077637,
-0.0236917492,
-0.0690521076,
-0.2420407981,
0.4297695458,
-0.4423103034,
-0.5072242022,
-0.4338136017,
0.2354564965,
0.0585268699,
-0.1644811779,
-0.3899994493,
0.3686197996,
-0.2135483921,
0.1273380816,
0.2562367618,
0.3571557701,
0.0298297834,
0.3313848078,
-0.4535017312,
-0.120437026,
0.7448206544,
-0.5261366367,
0.3190009892,
-0.2616108656,
0.3419782519,
0.4516818523,
-0.3614069223,
-0.501688242,
0.0079076402,
0.1330845207,
-0.0542377383,
-0.1616004705,
0.0992289111,
-0.3082869649,
0.1013124138,
-0.0707927942,
0.2413409948,
0.1822644621,
0.0541241616,
-0.0666988418,
-0.1139496863
]
|
https://github.com/huggingface/datasets/issues/1942 | [experiment] missing default_experiment-1-0.arrow | But no, since
`
metric = load_metric(metric_name)
`
is called for each process, the race condition is still there. So still getting:
```
ValueError: Error in finalize: another metric instance is already using the local cache file. Please specify an experiment_id to avoid colision between distributed metric instances.
```
i.e. the only way to fix this is to `load_metric` only for rank 0, but this requires huge changes in the code and all end users' code.
| the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you. | 76 | [experiment] missing default_experiment-1-0.arrow
the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you.
But no, since
`
metric = load_metric(metric_name)
`
is called for each process, the race condition is still there. So still getting:
```
ValueError: Error in finalize: another metric instance is already using the local cache file. Please specify an experiment_id to avoid colision between distributed metric instances.
```
i.e. the only way to fix this is to `load_metric` only for rank 0, but this requires huge changes in the code and all end users' code.
| [
-0.0743826106,
0.0189334024,
0.098625727,
0.2385593355,
0.0015654586,
0.0210894197,
0.1744566709,
0.2997217178,
0.3662588,
0.1215545982,
0.0272280686,
0.1309888959,
-0.3144579232,
-0.0137838349,
-0.0090083247,
-0.0354207493,
-0.0174289811,
-0.0118404869,
-0.2157492191,
-0.1407078505,
-0.3549722135,
0.4909446239,
-0.0940420181,
-0.0196760781,
-0.4025663137,
0.1862026155,
-0.1208147705,
0.1642288864,
-0.0712104961,
-0.6548621655,
0.3760331869,
0.211978063,
0.055022914,
0.5668933988,
-0.000122839,
-0.1647619158,
0.2224577963,
-0.1654274166,
-0.0895344839,
-0.2089777142,
0.0419953912,
-0.301823616,
0.4746407866,
-0.1672162861,
-0.0354674198,
-0.0663869902,
0.0834046081,
-0.4613866806,
0.4682588279,
-0.0254346766,
0.0765320659,
0.3800903261,
-0.1043441817,
-0.2316596508,
-0.049506858,
-0.1613815278,
0.0241746213,
0.4295057356,
-0.0905900374,
0.0036930905,
-0.3325565457,
0.2633510828,
0.1676269174,
0.0209395122,
0.2453758121,
-0.055975832,
0.0649289936,
-0.1188936979,
-0.105040811,
0.3209834397,
0.1520304978,
-0.1883414686,
-0.2298359573,
0.0978397578,
-0.1813545823,
-0.5551992655,
0.1535267383,
-0.0972424373,
0.0039797616,
0.0392490141,
-0.0380287543,
-0.0952161551,
-0.0568384714,
0.0566295907,
-0.1502936929,
0.0717538521,
-0.1363276392,
0.0569493435,
-0.0620995015,
0.2655830085,
-0.3586179912,
0.3487267494,
-0.0165709537,
0.1322407871,
-0.4776334763,
0.1030916646,
0.2333646566,
0.0192754865,
0.0638084039,
0.3710054457,
0.0047832155,
0.1104967892,
0.4419555664,
0.0989984721,
-0.0648538545,
0.4883598685,
0.2653137147,
-0.097263284,
0.1724759936,
0.1843726486,
-0.1093545705,
-0.3044107556,
0.1303543746,
-0.2305697948,
0.3590709269,
0.1428923458,
0.0501233302,
-0.4300653338,
0.0268947221,
0.3125571907,
-0.0175364707,
-0.1046422496,
0.1151105613,
0.3204921782,
0.0802920088,
0.0376733541,
0.2430389076,
0.3135304451,
-0.2338240892,
0.0523922853,
-0.4096280932,
-0.2092060596,
-0.0599776916,
0.3604541421,
0.0806869939,
-0.0867774412,
0.662260592,
-0.2452789098,
0.4363714159,
-0.0098047769,
0.204633832,
0.1801850498,
-0.0522348322,
0.3353194892,
0.0205430742,
0.2025181353,
0.4229382575,
0.1180681437,
-0.2378181368,
-0.222247839,
-0.1965863705,
-0.4890339375,
0.1327873617,
-0.0115740485,
-0.4982542098,
0.218323946,
0.2810570002,
0.2368828207,
-0.075057745,
-0.1865116507,
0.1740935147,
0.0632326975,
-0.1740944535,
-0.0775207207,
0.5013921261,
0.8000811934,
-0.3106374741,
-0.4090102315,
0.0621443093,
0.0198929645,
0.0597447865,
0.1834789515,
-0.0264083128,
0.112223208,
-0.239866972,
0.0430618301,
0.4500413537,
-0.3818282187,
-0.4404717088,
-0.0102440361,
-0.4845242798,
-0.0927991644,
0.1529064775,
0.0130150346,
0.1167050004,
0.1743210703,
0.059625417,
-0.2304721177,
0.1583908945,
-0.2232080549,
-0.4099035561,
-0.3159254193,
0.0827814266,
0.0175184458,
0.351108402,
0.0312786847,
-0.0216586236,
0.0502976216,
0.0938850641,
0.2171545923,
0.1900274009,
0.2495131791,
0.2989778519,
0.0074489759,
0.2794370651,
0.0626640022,
-0.1863882393,
0.3330546618,
-0.3847151399,
0.1210734248,
0.155823037,
0.0101861572,
-0.2554591894,
-0.2336650491,
-0.2074742764,
-0.3817096055,
0.0072998977,
-0.1816834211,
0.2119553685,
0.2074861228,
-0.2354958802,
0.1523828506,
0.0227622613,
0.2163389921,
-0.423148185,
0.0148605583,
-0.0297128465,
-0.3313263357,
-0.0202225298,
0.0606496297,
0.0434458293,
0.0521165058,
-0.207270354,
0.4505544305,
-0.2041246742,
0.2718487978,
0.3489958942,
0.1949973106,
-0.0731451139,
-0.3825898767,
-0.0086809378,
0.1035968587,
-0.0373467058,
0.0034667773,
-0.2115326673,
0.3656710982,
0.0740350038,
0.1468585581,
-0.351149112,
0.2701357305,
-0.0933459178,
-0.1494021565,
-0.5120648146,
-0.0564064384,
0.3746653199,
-0.2972073555,
0.3142364323,
-0.1348359138,
0.0955449045,
-0.0313752852,
0.2216872424,
0.2303459495,
0.1324338317,
0.0526791289,
-0.2068918645,
-0.0816361457,
0.0431524403,
-0.2377362847,
0.3779174984,
0.1831711084,
0.2262166142,
0.0800456479,
0.1042996496,
-0.1794140488,
0.0618311614,
0.0174043383,
-0.093975462,
0.155828163,
0.2129646987,
0.1486932635,
-0.3308373392,
-0.046664916,
-0.2257913053,
0.077769123,
-0.3252051771,
0.0463926718,
-0.1676026583,
0.3622935712,
0.1042041481,
0.0760789812,
-0.3205081522,
-0.200660944,
0.2137134075,
-0.1206796691,
0.055830311,
0.3729783595,
-0.3125833273,
0.5460640192,
0.1275181025,
-0.18352063,
-0.2804269195,
-0.2134809345,
-0.0769838616,
-0.1774238497,
-0.1685280502,
0.1996258348,
0.1353265196,
-0.1900619119,
0.0364902318,
0.0679251999,
-0.2923832238,
0.007269037,
0.0544488281,
0.600989759,
0.219851315,
0.1127616391,
-0.3618908226,
0.0661275089,
0.4660864174,
-0.4653952122,
-0.2988182306,
0.1108198389,
-0.0056102546,
0.2263548225,
-0.2566635609,
-0.303011179,
0.1503475159,
-0.3555740714,
0.6306395531,
0.0361630432,
0.0040373341,
-0.1828647107,
-0.0212516338,
0.1606978029,
-0.1455210447,
0.1835409105,
-0.3220936954,
-0.7370195985,
0.3779357076,
-0.0818956718,
-0.2262858897,
-0.0287558883,
0.1227795035,
0.3893445134,
-0.0262095034,
-0.5944405794,
-0.481017381,
0.0726499557,
0.2060049623,
0.0201440342,
0.0549135506,
0.5922465324,
-0.0786323249,
-0.003996334,
-0.0683099926,
-0.1145829707,
0.5944651365,
-0.1417895108,
0.1444730014,
-0.0960227475,
0.4891735911,
0.1768224388,
0.9024278522,
0.5595241189,
0.3208565414,
0.2859216332,
0.1434482485,
0.3294371963,
-0.0935018212,
-0.1837990582,
0.3400891125,
0.212522164,
-0.1073297709,
0.2406831086,
0.1164699942,
0.1155294105,
-0.1355737597,
-0.0306925643,
-0.2832494974,
-0.2555081248,
-0.1000094265,
-0.1111304834,
0.3113301694,
-0.0138610434,
-0.0408908986,
-0.2783925831,
0.0155793941,
0.4612798691,
0.4263333678,
0.2830249965,
0.2465914488,
-0.2043100744,
0.1160476059,
-0.60267061,
0.2750054598,
-0.4224064946,
0.0926005542,
-0.1565952599,
-0.2295764983,
0.0888544619,
-0.0187627748,
0.577018857,
0.107289955,
-0.1236118153,
0.0174962133,
-0.1422741115,
-0.4782926738,
-0.0497224256,
-0.0212904699,
-0.0193779171,
0.2601457834,
0.3804854155,
-0.4534815848,
-0.2462220341,
-0.0953103602,
-0.0955962539,
-0.3262086213,
-0.385243237,
-0.4633519948,
-0.0032967473,
-0.1292666942,
0.236742124,
-0.2321142107,
0.0642479509,
-0.0269821174,
0.0604758821,
-0.1549198925,
-0.0765354782,
0.0366818495,
0.129776895,
0.3405135572,
-0.2411498725,
0.2002918273,
0.5243802667,
-0.2138914317,
0.0506992415,
0.3819576204,
0.0767323673,
-0.0481710844,
0.3114058077,
-0.0057787932,
0.268409729,
0.5744401813,
-0.2220173627,
0.0661042854,
-0.0402307771,
0.3440594375,
-0.1733618379,
-0.0811488479,
0.2343105674,
-0.1451414078,
0.3937272727,
0.1732897758,
0.2727487981,
0.2667101622,
-0.1511026621,
0.3070549965,
-0.0249732397,
-0.0635656789,
0.338653177,
0.0756160766,
0.9486906528,
0.1284909248,
0.0594738796,
0.1395250261,
0.136572808,
0.3249999285,
0.0548073761,
0.1148585901,
-0.209852159,
-0.2724111676,
0.015761314,
-0.1055021808,
0.0776122063,
-0.2158324271,
-0.1212310791,
0.4221636653,
-0.3239581883,
-0.1714657992,
-0.2416451573,
-0.189141497,
-0.2325045615,
-0.222449109,
0.0643666685,
0.0192138366,
0.0016659965,
0.5368850231,
-0.2295256257,
-0.1801929027,
-0.2658548057,
-0.2863273323,
-0.0787068829,
-0.1080936342,
-0.2214948982,
-0.0420686379,
0.1932158619,
-0.1637639403,
-0.2138140202,
0.2345359176,
0.4904162586,
0.1107981727,
-0.3359956741,
-0.0386441313,
0.1185731068,
0.0472980402,
0.1092756465,
-0.0165890735,
0.1812066287,
-0.0596787371,
-0.0700884312,
0.0230900366,
-0.2034309506,
0.0294567589,
0.1439750493,
-0.1831578463,
-0.0728729516,
0.0270322803,
0.0658262372,
0.1261665225,
0.1493880749,
-0.2243225873,
0.0851812065,
0.2755258381,
-0.2201800048,
0.0531319156,
0.1045538634,
-0.2454174906,
-0.1072542295,
0.6161944866,
-0.1266842782,
-0.0828663185,
0.395639509,
-0.0293207522,
-0.028158145,
-0.0929279253,
0.0045105657,
0.47167781,
-0.7318611145,
0.0216785446,
-0.0043272958,
-0.018871462,
0.0596459322,
0.2084545046,
0.4314277172,
-0.1141747162,
-0.0196105596,
-0.1784308553,
-0.4242088795,
0.2923370004,
-0.2917726636,
0.1231567338,
-0.4502269328,
0.1341914088,
-0.183529824,
0.2939422131,
-0.263954103,
0.1568065286,
-0.2775065005,
-0.1364924312,
-0.1669441164,
-0.0555274747,
0.3321179748,
-0.0281809047,
-0.0911532417,
0.115694508,
-0.3989807069,
-0.0762548,
-0.2312701643,
0.1596203744,
-0.1257578731,
0.0551052317,
0.0076609803,
0.1867503077,
-0.073407501,
-0.1189984828,
0.0783569738,
0.2514327168,
0.0211226605,
0.0762161463,
0.2156768292,
0.183385551,
-0.1267374456,
0.1507463306,
0.1054923981,
-0.1982362419,
-0.1485911161,
-0.0037620582,
-0.3089410663,
-0.0378239863,
0.2045277357,
0.0469588675,
-0.1688237488,
0.1653287709,
0.094809778,
-0.0197557602,
-0.3375715911,
0.0840764046,
0.4339141846,
0.1084736511,
-0.1139043644,
0.0171609502,
-0.0484704562,
0.0941807628,
-0.154531166,
0.2720601857,
0.0388729833,
0.360687077,
0.0561299957,
0.2945480049,
0.2639492452,
-0.0546165779,
0.1945339292,
0.2769587338,
0.0772711113,
-0.1692988575,
0.3492944241,
-0.2080003172,
0.1232763305,
0.2905895412,
0.6146332026,
0.1501110941,
0.1940243989,
-0.1213557497,
-0.0478094704,
0.1758171171,
-0.1244243607,
0.3346287608,
-0.3251514733,
-0.1684166044,
-0.2493239641,
0.0356424451,
-0.0446150079,
-0.1554946154,
-0.4124147594,
0.6928098798,
-0.3596714437,
-0.3196225166,
-0.2396952063,
0.2137313634,
-0.1621915847,
-0.1622506231,
-0.1247980446,
-0.1749601662,
-0.1221316829,
-0.1295802444,
0.255628258,
-0.0027532869,
0.1339076757,
0.058440309,
-0.0129349213,
-0.1976455301,
-0.5636008382,
0.3360032439,
0.236990124,
-0.1671221107,
0.0380651429,
0.2539329231,
-0.3203012645,
0.0502870157,
0.6612638831,
0.1400392652,
0.0205173548,
-0.1053514779,
-0.006834913,
0.329179883,
0.0089210011,
-0.0917339325,
0.1179399043,
0.212398231,
-0.4631313086,
0.0149258571,
0.047355067,
-0.140191108,
0.4126123488,
-0.2860366404,
0.3127579987,
-0.2527724802,
0.4388634264,
-0.2093798667,
0.1386309564,
0.0330517739,
-0.2090283185,
-0.638252914,
0.2721563876,
-0.1211094782,
0.0055664335,
0.0730726495,
-0.3161961734,
0.0315935463,
-0.0653401911,
0.1874741018,
0.3362929225,
-0.0472614765,
-0.3999143839,
-0.3157867789,
-0.6288838983,
0.3125654161,
0.1188523248,
0.3920561969,
0.0013996661,
0.3996368945,
-0.1393110156,
0.1077264398,
0.0806470364,
0.011525006,
-0.108497709,
0.0430236496,
-0.5370867848,
0.2043399662,
-0.1205614135,
-0.0920397937,
0.080926083,
0.2269993275,
0.0197599214,
-0.1926590055,
-0.0032362267,
0.2365330458,
-0.1842131764,
-0.0328188241,
0.2254677713,
0.3922174871,
0.0917387605,
-0.0026018233,
0.0817169398,
-0.038261883,
-0.2293898612,
-0.1227652878,
-0.1318762302,
-0.0989051685,
-0.3089472353,
0.4359291196,
-0.3517574966,
-0.4784052968,
-0.3654706776,
0.2429838628,
0.0612318069,
-0.2057875097,
-0.2005639374,
0.2870569527,
-0.0907273591,
0.0183587391,
0.2171037346,
0.3107778728,
0.0868569613,
0.3682159781,
-0.3748181462,
-0.1778045446,
0.7422965765,
-0.5126376152,
0.1951847821,
-0.2612096369,
0.4384709001,
0.476701349,
-0.3749294281,
-0.3257742822,
-0.0008096234,
0.1407494098,
0.0945584103,
-0.1339271963,
0.1337973028,
-0.4526486099,
-0.0406460017,
-0.0503083803,
0.1235828549,
0.1436061114,
0.0090165799,
0.0242892094,
-0.1226511598
]
|
https://github.com/huggingface/datasets/issues/1942 | [experiment] missing default_experiment-1-0.arrow | OK, here is a workaround that works. The onus here is absolutely on the user:
```
diff --git a/examples/seq2seq/run_seq2seq.py b/examples/seq2seq/run_seq2seq.py
index 2a060dac5..c82fd83ea 100755
--- a/examples/seq2seq/run_seq2seq.py
+++ b/examples/seq2seq/run_seq2seq.py
@@ -520,7 +520,11 @@ def main():
# Metric
metric_name = "rouge" if data_args.task.startswith("summarization") else "sacrebleu"
- metric = load_metric(metric_name)
+ import torch.distributed as dist
+ if dist.is_initialized():
+ metric = load_metric(metric_name, num_process=dist.get_world_size(), process_id=dist.get_rank())
+ else:
+ metric = load_metric(metric_name)
def postprocess_text(preds, labels):
preds = [pred.strip() for pred in preds]
@@ -548,12 +552,17 @@ def main():
# Some simple post-processing
decoded_preds, decoded_labels = postprocess_text(decoded_preds, decoded_labels)
+ kwargs = dict(predictions=decoded_preds, references=decoded_labels)
+ if metric_name == "rouge":
+ kwargs.update(use_stemmer=True)
+ result = metric.compute(**kwargs) # must call for all processes
+ if result is None: # only process with rank-0 will return metrics, others None
+ return {}
+
if metric_name == "rouge":
- result = metric.compute(predictions=decoded_preds, references=decoded_labels, use_stemmer=True)
# Extract a few results from ROUGE
result = {key: value.mid.fmeasure * 100 for key, value in result.items()}
else:
- result = metric.compute(predictions=decoded_preds, references=decoded_labels)
result = {"bleu": result["score"]}
prediction_lens = [np.count_nonzero(pred != tokenizer.pad_token_id) for pred in preds]
```
This is not user-friendly to say the least. And it's still wasteful as we don't need other processes to do anything.
But it solves the current race condition.
Clearly this calls for a design discussion as it's the responsibility of the Trainer to handle this and not user's. Perhaps in the `transformers` land? | the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you. | 233 | [experiment] missing default_experiment-1-0.arrow
the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you.
OK, here is a workaround that works. The onus here is absolutely on the user:
```
diff --git a/examples/seq2seq/run_seq2seq.py b/examples/seq2seq/run_seq2seq.py
index 2a060dac5..c82fd83ea 100755
--- a/examples/seq2seq/run_seq2seq.py
+++ b/examples/seq2seq/run_seq2seq.py
@@ -520,7 +520,11 @@ def main():
# Metric
metric_name = "rouge" if data_args.task.startswith("summarization") else "sacrebleu"
- metric = load_metric(metric_name)
+ import torch.distributed as dist
+ if dist.is_initialized():
+ metric = load_metric(metric_name, num_process=dist.get_world_size(), process_id=dist.get_rank())
+ else:
+ metric = load_metric(metric_name)
def postprocess_text(preds, labels):
preds = [pred.strip() for pred in preds]
@@ -548,12 +552,17 @@ def main():
# Some simple post-processing
decoded_preds, decoded_labels = postprocess_text(decoded_preds, decoded_labels)
+ kwargs = dict(predictions=decoded_preds, references=decoded_labels)
+ if metric_name == "rouge":
+ kwargs.update(use_stemmer=True)
+ result = metric.compute(**kwargs) # must call for all processes
+ if result is None: # only process with rank-0 will return metrics, others None
+ return {}
+
if metric_name == "rouge":
- result = metric.compute(predictions=decoded_preds, references=decoded_labels, use_stemmer=True)
# Extract a few results from ROUGE
result = {key: value.mid.fmeasure * 100 for key, value in result.items()}
else:
- result = metric.compute(predictions=decoded_preds, references=decoded_labels)
result = {"bleu": result["score"]}
prediction_lens = [np.count_nonzero(pred != tokenizer.pad_token_id) for pred in preds]
```
This is not user-friendly to say the least. And it's still wasteful as we don't need other processes to do anything.
But it solves the current race condition.
Clearly this calls for a design discussion as it's the responsibility of the Trainer to handle this and not user's. Perhaps in the `transformers` land? | [
-0.0640709698,
-0.0787639916,
0.0965642631,
0.2534792721,
-0.0056876931,
-0.0212870073,
0.3123221099,
0.2638015151,
0.211024344,
0.2581809461,
0.0451733731,
0.3624680638,
-0.352606833,
-0.1786396056,
0.0343574323,
-0.0347166024,
-0.044143755,
-0.1339791268,
-0.358322978,
-0.12399441,
-0.1907990426,
0.4987674952,
-0.0344166979,
-0.0297839325,
-0.3931264877,
0.2241770327,
-0.1640089899,
0.1788710803,
0.064350538,
-0.5618360639,
0.3226026893,
0.1360853761,
0.0452937447,
0.5764807463,
-0.0001236324,
-0.1678351015,
0.1872866899,
-0.2450377643,
-0.1874422729,
-0.2717464566,
0.227120012,
-0.3114354908,
0.4968201518,
-0.2364774048,
-0.1577861756,
0.0378682278,
-0.0054119467,
-0.3434018493,
0.423699528,
0.0571900792,
0.0859313756,
0.3330074251,
-0.0323254578,
-0.1901234537,
0.1212336496,
-0.0653861091,
-0.0368984453,
0.3913043737,
-0.0438003838,
0.0700678676,
-0.2391370684,
0.299698025,
0.1755197346,
-0.0402258709,
0.3147487938,
-0.0494451262,
-0.2011663467,
-0.0775261447,
-0.1052094325,
0.3080209196,
0.2359144539,
-0.2234062403,
-0.237583518,
0.0531367324,
-0.1129200757,
-0.3445172012,
0.1445093453,
-0.0051628966,
-0.0187257007,
0.0235214755,
0.0591397174,
-0.1390451938,
-0.0627026185,
0.0998460278,
-0.0452244245,
0.013915739,
-0.1335917562,
0.105486773,
-0.0017542032,
0.1352349371,
-0.3275973499,
0.3069051504,
0.0391774997,
0.1289856136,
-0.3677837849,
0.1243337542,
0.2337085456,
0.0210838821,
0.0918097347,
0.4560724497,
0.0586394966,
0.0463431738,
0.4054610431,
0.1031669378,
-0.1804310828,
0.491076827,
0.2882264853,
0.1206451356,
0.1309710592,
0.2508909702,
-0.0607218817,
-0.294939518,
0.1342704743,
-0.258698076,
0.2855695188,
0.2531367242,
0.0631744936,
-0.4151258767,
0.046472203,
0.1691829115,
-0.0770842284,
-0.1774358451,
0.1314894855,
0.4097200334,
0.1488400549,
0.0084702047,
0.3483397961,
0.3792476356,
-0.1620043665,
0.0054708361,
-0.3775833249,
-0.161901623,
-0.0832367912,
0.3270739019,
0.1530537903,
-0.1119456068,
0.6152181029,
-0.2334932238,
0.4344536066,
-0.0676148906,
0.208604753,
0.2173029035,
0.0877950117,
0.1757380366,
-0.0002522216,
0.1726160496,
0.349971503,
0.3042707443,
-0.2953211367,
-0.1659080237,
-0.1075638607,
-0.5697391033,
0.1044742838,
-0.0256731324,
-0.3686074018,
0.2524024546,
0.3646578789,
0.1765206903,
-0.0878764316,
-0.1766688228,
0.2183124572,
0.0424640849,
-0.1899274141,
-0.1322889924,
0.5401469469,
0.7955898643,
-0.2234707475,
-0.3928964436,
0.0861315951,
-0.0302690528,
-0.0688861385,
0.1711060852,
-0.1304829419,
0.1734696031,
-0.3124315739,
0.0132101914,
0.4526492357,
-0.4541598558,
-0.5230979919,
-0.0551198013,
-0.3473707438,
-0.1022440791,
0.1810641587,
0.0192642193,
0.1447809637,
0.1427843124,
0.1297153831,
-0.145538643,
0.2052160501,
-0.2678941786,
-0.3874984086,
-0.2713223696,
0.0696764886,
-0.0526347235,
0.2598972619,
0.0193603914,
0.0312665924,
-0.0005676819,
0.1223187894,
0.1125743762,
0.153045103,
0.3094590604,
0.1673349738,
0.1094969958,
0.227171436,
0.0797587931,
-0.2535488307,
0.2806078792,
-0.2641235888,
0.0964866951,
0.054757122,
-0.0591280386,
-0.2017364055,
-0.1917340308,
-0.118976675,
-0.4788511395,
-0.0093121827,
0.0025924875,
0.1778896004,
0.0980060771,
-0.0833217502,
0.0272524133,
0.0022780551,
0.2093143463,
-0.4971334934,
0.0486031249,
-0.1358311176,
-0.3562149107,
-0.0538285933,
0.1624727994,
0.082434848,
0.0275182202,
-0.2172622532,
0.414255023,
-0.2254361063,
0.2963484824,
0.2505710423,
0.0866477564,
-0.1558145285,
-0.4436958432,
0.0706842542,
0.1534369886,
-0.0243407115,
0.0595748946,
-0.2230675966,
0.4005185962,
0.0576048531,
0.088164486,
-0.3915156424,
0.3206072748,
-0.062292695,
-0.1256734878,
-0.5161962509,
-0.0653316006,
0.4644509852,
-0.2338354439,
0.3068930209,
-0.1485266834,
-0.1147532612,
0.0304503981,
0.0552104414,
0.1526013315,
0.2161271721,
0.049204383,
-0.210638687,
0.0539288856,
0.0803138092,
-0.2700402141,
0.4026062787,
0.2301346213,
0.2446661741,
0.0396016613,
0.2069370002,
-0.1649564058,
0.0762508735,
-0.0633523166,
-0.1690060496,
0.1789561212,
0.299423039,
0.1694059223,
-0.2687005997,
-0.0116005419,
-0.3858015835,
-0.0177339297,
-0.3161156774,
0.108588472,
-0.1061812416,
0.3600184619,
0.0628942177,
0.0278819427,
-0.2497001588,
-0.2115065157,
0.217203021,
-0.1287646741,
-0.0082144672,
0.3403944373,
-0.143834427,
0.4247651398,
0.1005565301,
-0.2894515693,
-0.2572137713,
-0.1998004764,
-0.0760464966,
-0.1793351471,
-0.2656765878,
0.045699995,
0.2180574089,
-0.1610603631,
0.0553274862,
-0.1083597764,
-0.2996194065,
0.0607738979,
0.0864420831,
0.5465068221,
0.2948963642,
0.0005502215,
-0.1870800704,
0.0301563237,
0.4708813429,
-0.4639459252,
-0.2469665408,
0.0697956234,
0.0687670931,
0.3005346358,
-0.302826941,
-0.410823971,
0.0369479507,
-0.4303353429,
0.6223108768,
0.0505605415,
0.0192916002,
-0.2766924798,
0.0470694937,
0.1442531645,
-0.1902222931,
0.3671060801,
-0.3098248243,
-0.6642646194,
0.4911519885,
-0.1532042921,
-0.2969999909,
-0.0493566506,
0.0273513794,
0.4338548779,
0.0113270944,
-0.6472851038,
-0.4276648462,
0.0734203458,
0.3311349154,
-0.0820798427,
0.1234309897,
0.5292959809,
-0.1036515757,
0.0432881974,
-0.0859868005,
-0.1932816654,
0.4761615694,
-0.2479906082,
0.2904287875,
-0.0502363779,
0.471001327,
0.2042385936,
1.0362510681,
0.4318810999,
0.1980847865,
0.2408501357,
0.0621299855,
0.3678742349,
-0.1329011917,
-0.2530630529,
0.3594071567,
0.2157834172,
-0.0651655793,
0.1750032902,
0.140112415,
0.1193041876,
-0.1175365001,
-0.1354065239,
-0.3230400085,
-0.3765502274,
0.058439143,
-0.3119448125,
0.2293656021,
-0.1024173498,
0.1097868234,
-0.2834073603,
-0.0170252956,
0.4524647593,
0.3811737597,
0.2687610984,
0.2686800361,
-0.1061638296,
0.1557907462,
-0.6143527627,
0.319568336,
-0.4150725901,
0.0204217955,
-0.1964595169,
-0.2585926652,
0.1327745765,
0.0286133979,
0.570192337,
0.1704188585,
-0.0772310123,
-0.0223531928,
-0.2900950313,
-0.5728945732,
-0.1306664348,
0.033599522,
0.0039161504,
0.3430419862,
0.2983965576,
-0.4971287847,
-0.2174242586,
-0.1325905919,
-0.0143526504,
-0.294871062,
-0.2986007035,
-0.3033484221,
0.0376956053,
-0.3638683558,
0.125795573,
-0.1481667161,
0.0019770374,
0.0124047911,
0.0938544124,
-0.1730408072,
-0.033301387,
-0.0419655442,
0.0819391906,
0.4452997148,
-0.3680723011,
0.2138538212,
0.4355838299,
-0.0814055055,
0.0465964042,
0.4527382255,
0.0202474799,
-0.032204181,
0.2494156808,
-0.1702364981,
0.1760367155,
0.56836766,
-0.2360544354,
-0.006551974,
-0.168375507,
0.3352351487,
-0.1813476831,
-0.0947213992,
0.2148927152,
-0.1399390846,
0.4028425217,
0.0797778741,
0.1491571367,
0.2951567471,
-0.1458464116,
0.3089534044,
-0.0896982998,
-0.1584065855,
0.3474823236,
0.028355917,
1.0431011915,
0.1858013272,
0.1939707547,
0.0941930264,
0.059487436,
0.3286901712,
0.0322481021,
0.042359639,
-0.2455369383,
-0.1855899096,
0.0552307107,
-0.1338484287,
-0.0892543271,
-0.2128922343,
-0.2421694845,
0.4602893591,
-0.3985318542,
-0.11877691,
-0.2751250565,
-0.2161845118,
-0.3653043211,
-0.154029116,
0.1623810083,
0.0106244162,
0.0389809757,
0.5765023828,
-0.2063028812,
-0.1709362417,
-0.3550461531,
-0.4208045602,
-0.1701824218,
-0.0027209513,
-0.2345435172,
0.0126434378,
0.2596082985,
-0.3003924489,
-0.1275202185,
0.3978984654,
0.375356555,
0.1119138971,
-0.2954159677,
-0.0671835914,
0.0727767125,
0.0780793056,
-0.0095543237,
-0.010505992,
0.2022233456,
-0.0534296297,
-0.0567146204,
0.0370838493,
-0.1553321481,
0.0644345284,
0.0799803957,
-0.104557924,
-0.0424971543,
-0.1324029118,
0.0671941712,
0.1465180963,
0.0625551268,
-0.2441197336,
0.0631632954,
0.3170137107,
-0.3118458092,
-0.0164041761,
0.13000305,
-0.1914991438,
-0.0526278242,
0.6006054878,
-0.2180668712,
-0.0574903749,
0.4439827502,
0.0863895416,
-0.0900120065,
-0.0896647274,
-0.0095597273,
0.4702002406,
-0.8980284929,
0.063202709,
-0.0824750736,
-0.0375499614,
0.0195322726,
0.21734716,
0.3759931326,
-0.0564709976,
-0.0513749532,
-0.1332806796,
-0.4421546757,
0.249110207,
-0.2822007835,
0.2810610831,
-0.3498195708,
0.0752844736,
-0.1540610939,
0.2654629946,
-0.2644132674,
0.1684679836,
-0.1766667515,
-0.0392873734,
-0.0815751851,
0.0030674511,
0.3155995607,
-0.018136017,
-0.0913405046,
0.1194008216,
-0.3476512432,
-0.0852038562,
-0.205667004,
0.1578653306,
-0.1684209108,
0.1116117686,
-0.126875937,
0.1770161837,
0.0223470517,
-0.1894457489,
0.1027839482,
0.2703881264,
0.0493440256,
0.2141863555,
0.1147245169,
0.2388999909,
-0.0491267666,
0.2069228142,
0.0891238451,
-0.0931500569,
-0.1271403134,
0.0269982331,
-0.3199724555,
-0.0794885084,
0.1768061966,
0.0992084146,
-0.0745758489,
0.2261695713,
0.0143871605,
0.0950904116,
-0.3218648434,
-0.083181724,
0.3944783509,
0.025238879,
-0.224036783,
0.0014872923,
-0.0390670002,
0.0907803252,
-0.0961547047,
0.2872498035,
-0.0495018288,
0.2823805213,
0.0991163626,
0.2836002111,
0.2086954564,
-0.0566606075,
0.0748733878,
0.1841033995,
-0.0485473052,
-0.1143179834,
0.387547195,
-0.0666377544,
0.0531389751,
0.2888017893,
0.5470502377,
0.1314864159,
0.2106274664,
-0.1264865696,
-0.062876001,
0.358679831,
-0.0406755768,
0.2327470928,
-0.0682585612,
-0.1420317292,
-0.1085528955,
-0.1151633486,
0.1268030107,
-0.2630986571,
-0.38830024,
0.69059515,
-0.1910870075,
-0.2771377563,
-0.0456670709,
0.2752058208,
-0.1335231662,
-0.1646233499,
-0.1777417362,
-0.1472783387,
-0.1358103752,
-0.1351953,
0.252479881,
-0.0429370143,
0.175387755,
0.1447415948,
0.0329225138,
-0.2331385612,
-0.3482341468,
0.2942015827,
0.1979301721,
-0.1560440063,
-0.0297631528,
0.2149162441,
-0.2448701859,
0.140407443,
0.5754086375,
0.2674568295,
-0.0427275896,
-0.058017429,
0.088527739,
0.2895347178,
0.0024859668,
-0.1617274433,
0.0645728037,
0.1674533933,
-0.414164871,
0.0965313166,
0.0538549609,
-0.1895056814,
0.413664937,
-0.2827783525,
0.2279515415,
-0.1521188766,
0.4434225261,
-0.2251651734,
0.1118195057,
-0.0354709029,
-0.1718774736,
-0.6200803518,
0.2504793406,
-0.1109715477,
0.0327469744,
0.0921415761,
-0.2865738273,
0.0349233188,
-0.081704013,
0.1180626377,
0.3765929043,
0.0629151091,
-0.3114416003,
-0.2652312517,
-0.5255098939,
0.3029746711,
0.2050181329,
0.2848009467,
-0.0820880681,
0.3575697243,
-0.0763022751,
0.110862352,
0.2054357976,
-0.0856378302,
0.0611879006,
0.1663653255,
-0.5453773737,
0.0388830937,
-0.1388043612,
-0.0357953496,
0.1995833516,
0.2482260615,
-0.2193720788,
-0.1935057789,
0.0216775239,
0.165612027,
-0.3292374313,
-0.1445856243,
0.2926346064,
0.4417030215,
0.0879333168,
0.0814846829,
0.0020327808,
-0.0957132131,
-0.190130055,
-0.1178384349,
-0.0816708356,
-0.1169572696,
-0.2638620138,
0.3734413981,
-0.3695464134,
-0.4859572053,
-0.4547895193,
0.2592763603,
0.0549926795,
-0.122169517,
-0.204932183,
0.3347382247,
-0.1531440169,
0.0585969165,
0.1520989388,
0.3737300932,
0.0079820678,
0.4060662985,
-0.4049216211,
-0.1603152305,
0.7884656787,
-0.4327734113,
0.2788587809,
-0.2969712913,
0.3566491306,
0.4510628283,
-0.3289367855,
-0.4853737056,
0.0553710721,
0.1302497089,
0.0357774533,
-0.0032266222,
0.0996123329,
-0.3638700843,
0.0264711343,
-0.080309093,
0.1829399914,
0.184614867,
0.068811655,
-0.0152423568,
-0.1749409139
]
|
https://github.com/huggingface/datasets/issues/1942 | [experiment] missing default_experiment-1-0.arrow | I don't see how this could be the responsibility of `Trainer`, who hasn't the faintest idea of what a `datasets.Metric` is. The trainer takes a function `compute_metrics` that goes from predictions + labels to metric results, there is nothing there. That computation is done on all processes
The fact a `datasets.Metric` object cannot be used as a simple compute function in a multi-process environment is, in my opinion, a bug in `datasets`. Especially since, as I mentioned before, the multiprocessing part of `datasets.Metric` has a deep flaw since it can't work in a multinode environment. So you actually need to do the job of gather predictions and labels yourself.
The changes you are proposing Stas are making the code less readable and also concatenate all the predictions and labels `number_of_processes` times I believe, which is not going to make the metric computation any faster.
| the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you. | 144 | [experiment] missing default_experiment-1-0.arrow
the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you.
I don't see how this could be the responsibility of `Trainer`, who hasn't the faintest idea of what a `datasets.Metric` is. The trainer takes a function `compute_metrics` that goes from predictions + labels to metric results, there is nothing there. That computation is done on all processes
The fact a `datasets.Metric` object cannot be used as a simple compute function in a multi-process environment is, in my opinion, a bug in `datasets`. Especially since, as I mentioned before, the multiprocessing part of `datasets.Metric` has a deep flaw since it can't work in a multinode environment. So you actually need to do the job of gather predictions and labels yourself.
The changes you are proposing Stas are making the code less readable and also concatenate all the predictions and labels `number_of_processes` times I believe, which is not going to make the metric computation any faster.
| [
-0.0986770317,
0.087464869,
0.0754723325,
0.1241441593,
0.1474276036,
-0.0701842383,
0.3525935709,
0.2356341928,
0.1611240059,
0.2098140866,
0.0004215879,
0.214056015,
-0.3455355763,
0.1935885996,
0.0740702897,
-0.0832459331,
-0.0075624692,
-0.1163946018,
-0.2869691551,
0.0001237781,
-0.2612045705,
0.4958017468,
-0.1959235817,
0.1165344492,
-0.4939330518,
0.0160645358,
-0.1405586153,
0.0436574966,
-0.1571672261,
-0.6827937961,
0.177974537,
0.2850544751,
0.0590011328,
0.6136052608,
-0.0001197262,
-0.1275454313,
0.1432345212,
-0.0766493604,
-0.1142279357,
-0.1018685475,
0.0062035383,
-0.3750231862,
0.5041077733,
-0.2027845383,
-0.0246382132,
-0.1871018112,
-0.0009075949,
-0.3866353333,
0.4851271808,
0.1960164905,
0.1148971021,
0.3724756539,
-0.0633554384,
-0.2893131971,
-0.0304379202,
-0.1797465384,
-0.0004409851,
0.306309104,
-0.118035078,
0.1041779295,
-0.2638342977,
0.2987608314,
0.1747553796,
-0.1579255909,
0.3985741138,
0.0108561749,
0.0237378776,
-0.0750848427,
-0.0263445489,
0.2280960381,
0.3640277684,
-0.2081998736,
-0.4027554393,
0.0213227607,
-0.1136106029,
-0.4449653625,
0.0867720619,
-0.1544952691,
-0.1124911308,
0.0173525624,
-0.0512010083,
-0.091870077,
-0.1205046624,
-0.1065767631,
-0.1400079876,
0.0415048786,
-0.1515363902,
0.1079932451,
0.0986205116,
0.29403916,
-0.2250005007,
0.4625897408,
0.1078086793,
0.0874906033,
-0.5861874819,
0.0515590608,
0.1689260155,
-0.1378932297,
0.0261833668,
0.3476355076,
0.1393158436,
0.1647455692,
0.4749912024,
0.0469468795,
-0.0859803706,
0.4959521294,
0.1158405095,
-0.0413894951,
0.2166434675,
0.2328992337,
-0.1324020624,
-0.3073427081,
0.1509370804,
-0.28944695,
0.407508105,
0.1650962085,
-0.0240274556,
-0.3971653879,
0.0111140739,
0.232166186,
-0.1615177244,
-0.3076353669,
0.1635745168,
0.3015664518,
-0.0188989919,
0.1546542048,
0.2649488151,
0.2677026689,
-0.2153813094,
0.0336755849,
-0.362700671,
-0.1388625205,
-0.2221656293,
0.5311322808,
-0.0336128734,
-0.1031349301,
0.4908149838,
-0.2240336388,
0.3613180518,
-0.1143148467,
0.1868816018,
0.1512250304,
0.072925739,
0.1448818445,
-0.0949602574,
0.0191625673,
0.1993147135,
-0.0007017746,
-0.2345670313,
-0.3136923909,
-0.1904587895,
-0.5587620139,
0.0460782424,
0.0113417897,
-0.4121507406,
0.1687011421,
0.1926000118,
0.439054966,
-0.0838325769,
-0.0827373937,
0.1543188542,
-0.0658368245,
-0.2503940463,
-0.0533928759,
0.5387085676,
0.6572610736,
-0.2708116174,
-0.3530546129,
0.1573345959,
-0.0764825493,
0.0305979345,
0.186612457,
-0.0675415695,
0.33159706,
-0.1351322234,
0.0338992886,
0.3187025487,
-0.3188117146,
-0.2820300162,
-0.1126638129,
-0.2808203399,
-0.0668344721,
0.1820888668,
-0.0332549289,
0.2036235332,
0.1777173281,
0.0793950707,
-0.2812538743,
0.1992525309,
-0.2796543539,
-0.3488395214,
-0.2571009994,
-0.0160809979,
0.0350258201,
0.4142493606,
0.1447870731,
-0.0155535797,
0.2191105932,
0.0989588946,
0.0124073364,
0.1865233034,
0.1369632334,
0.2173771113,
0.0301678311,
0.2122124434,
-0.0668712705,
-0.259488374,
0.1811954081,
-0.2249533832,
0.2644389868,
0.181270346,
-0.1109846681,
-0.1313762963,
-0.0319106206,
-0.0450460166,
-0.3800313175,
0.0381470434,
0.0193698611,
0.2461987734,
0.0636057183,
-0.1863694489,
0.2601462603,
-0.0229208469,
0.1970862448,
-0.307703048,
0.0054115532,
-0.049326349,
-0.2693817616,
-0.0472343564,
0.2843675911,
0.013506854,
0.0620761663,
-0.1563109159,
0.4471608996,
-0.097178258,
0.1208166257,
0.2460495383,
0.2522507608,
-0.100149177,
-0.2472558469,
0.0162443351,
-0.0177390147,
0.0309684109,
-0.0384249277,
-0.186736986,
0.4048729837,
-0.0063227159,
0.1802694947,
-0.1444067657,
0.2286260277,
-0.1040407196,
-0.014684326,
-0.6104432344,
-0.0441227071,
0.181778565,
-0.3588337302,
0.285474062,
-0.073217012,
-0.1879294962,
0.0873121545,
0.2204129696,
0.0784532204,
-0.0388828106,
0.1840816736,
-0.1221865267,
-0.1293783635,
-0.0313922241,
-0.0752814338,
0.437256813,
0.1981726289,
0.2658682764,
0.1368625909,
0.1789864302,
-0.2482044846,
0.1564262211,
0.1467078477,
-0.198352918,
0.0213169567,
0.1952257901,
0.1843654513,
-0.2286498398,
-0.1335853487,
-0.2828975618,
0.0912204012,
-0.3479498029,
0.111481756,
-0.1617620885,
0.3325735033,
-0.025605388,
0.0918038711,
-0.18454808,
-0.2695584893,
0.2477205843,
-0.1749941558,
-0.0806615725,
0.3019291759,
-0.1997396797,
0.5645968914,
0.0458002687,
-0.054394003,
-0.1539079696,
-0.1289449036,
0.0248095561,
-0.1263793558,
-0.1107393727,
0.2380955964,
0.1443099529,
-0.1409783065,
0.0804135799,
-0.1126382798,
-0.3777039349,
0.0318098031,
-0.0719137043,
0.6136100292,
0.3686547875,
-0.0671829805,
-0.3057754636,
0.0558077283,
0.4334177077,
-0.4616743624,
-0.1821401417,
-0.0158739146,
0.0210347828,
0.1119773537,
-0.228441298,
-0.4090144038,
-0.0333865881,
-0.3924376369,
0.6301874518,
-0.030286558,
0.0650706887,
-0.1423581541,
0.0048807417,
0.3127193749,
-0.2132499367,
0.285561651,
-0.3027740717,
-0.6883825064,
0.3615091145,
-0.1526073813,
-0.278452605,
-0.0922786742,
0.1246673986,
0.4610550404,
-0.1010716036,
-0.6390308738,
-0.4874394536,
-0.0583329983,
0.1837804914,
-0.085485585,
0.1019594893,
0.5934663415,
0.0402630121,
-0.1249963716,
-0.0401613414,
-0.3275841475,
0.6002624631,
-0.2267068774,
-0.0799338743,
-0.0480874553,
0.3807921112,
0.1421982646,
0.9527531266,
0.5312840343,
0.0835817307,
0.2418910116,
0.0609008148,
0.4276575446,
-0.1242133155,
-0.2087624073,
0.3594118059,
0.0553972013,
-0.0696332306,
0.2196853012,
0.075375326,
0.2284237444,
-0.0930810496,
0.0747020394,
-0.1595892161,
-0.3905132413,
0.0516242124,
-0.0709964782,
0.2339845598,
-0.0320988335,
0.0994046628,
-0.2225833237,
0.0105919773,
0.3239011168,
0.3276200891,
0.2672767043,
0.177355513,
-0.402730763,
-0.0141267646,
-0.6917688251,
0.2999632955,
-0.3306576014,
0.055032827,
-0.1726820469,
-0.3012735248,
0.1569829732,
-0.00326484,
0.5102086663,
0.167026177,
-0.3256073892,
0.0979514793,
-0.2732121348,
-0.351805687,
-0.032237459,
-0.1057448089,
-0.0924542546,
0.1303735226,
0.4627732337,
-0.3822813332,
-0.4021390676,
-0.057543382,
-0.0055290279,
-0.2024351507,
-0.2122658789,
-0.2753814161,
-0.190608725,
-0.1053454652,
0.2593513727,
-0.1286328584,
0.1493755281,
-0.0751165748,
0.0105618872,
-0.1109575704,
0.0294923112,
0.0498247966,
0.2006752193,
0.3328443468,
-0.2227475047,
0.3211789131,
0.5052852035,
0.0824889839,
0.1085559428,
0.3950393498,
-0.0712282881,
-0.0762939453,
0.2625710666,
-0.0787665471,
0.3977540433,
0.5973266959,
-0.3462332785,
0.0504738018,
0.001816199,
0.4982073307,
-0.1901553571,
0.0729651749,
0.2902303934,
0.0637290478,
0.3196472526,
0.0237538889,
0.2319912612,
0.3206913173,
-0.2400918901,
0.1433272511,
0.0838350356,
-0.2308395952,
0.2619360983,
0.1453871429,
0.9682908654,
0.0424494706,
0.1907758117,
0.2242966294,
0.0791934431,
0.4160703123,
0.0028478613,
0.0480358899,
-0.2100679278,
-0.3447067738,
0.0477676392,
-0.0816002265,
0.002189744,
-0.2576919794,
-0.3582122922,
0.3531534076,
-0.1213832274,
-0.0985355303,
-0.2188066691,
-0.032966435,
-0.0591556132,
-0.3148885369,
0.0249198545,
0.0341159031,
0.0702984482,
0.2626045644,
-0.1506990939,
-0.1671605855,
-0.2209926993,
-0.2636075616,
-0.1547156125,
-0.2230546474,
-0.2821092606,
-0.1693058014,
0.2403293699,
-0.0131512834,
0.0276421458,
0.2988246083,
0.4105800688,
0.0932677016,
-0.3142994046,
-0.1085324585,
-0.1605775207,
0.213030532,
0.1821777374,
-0.0810614899,
0.279546082,
-0.1318838,
-0.0807681605,
-0.0057211327,
-0.2108217329,
0.0140889389,
0.0495154709,
-0.1452659816,
-0.0465457626,
-0.1562492102,
0.1377625018,
0.0298857465,
-0.0652444586,
-0.3307301402,
0.0995554104,
0.2345897555,
-0.2563970089,
0.2924689353,
0.1632493883,
-0.2699543536,
-0.0011783644,
0.4867063165,
-0.0743929595,
-0.046913825,
0.534265697,
0.041793678,
-0.0784231275,
-0.1546751708,
-0.0377025567,
0.3748118877,
-0.6188098192,
0.2553613484,
-0.0921283737,
-0.1034340113,
0.0324723311,
0.2574296296,
0.2556150854,
-0.1610132009,
-0.051172331,
-0.1950923651,
-0.3997429609,
0.3095517159,
-0.2061041445,
0.2349152565,
-0.4314936996,
0.2607111335,
-0.1639533937,
0.2664734721,
-0.3096978366,
0.0712419301,
-0.3173696101,
0.004819206,
-0.0426048823,
-0.0775401071,
0.354088366,
0.0196156725,
-0.0791098773,
0.1467722356,
-0.341951102,
-0.122780785,
-0.2055004686,
0.1432721466,
-0.1030792892,
0.0037044084,
0.1053149551,
0.2841439545,
-0.2889699042,
-0.2163694203,
0.1446666867,
0.2201307416,
-0.0803181455,
0.1649865955,
0.02273711,
0.1731154025,
0.0217941906,
0.1275439411,
0.3193960786,
-0.1167130917,
-0.255214572,
0.1674157232,
-0.3102364242,
-0.1582752764,
0.2583625913,
0.2446473688,
-0.0530162118,
0.247622177,
-0.0005027817,
0.0390849821,
-0.4149768949,
0.0152036948,
0.5152156949,
0.211312294,
0.0148272486,
-0.0132395206,
0.0306371078,
0.0629688054,
-0.2263820171,
0.2248075902,
-0.0241946522,
0.1465189457,
0.1827188581,
0.3415965736,
0.2004184574,
-0.0087897805,
0.1131297573,
0.1668819189,
-0.0762682632,
-0.0972016156,
0.3929454088,
-0.0642777532,
0.0135833332,
0.3271149695,
0.6098667383,
0.0715300292,
0.1015051231,
0.1042543352,
-0.115217112,
0.319286108,
-0.0454615504,
0.3231513202,
-0.196245864,
-0.0204827636,
-0.1880554557,
0.102782324,
0.0078949407,
-0.2648671269,
-0.4510086775,
0.760166347,
-0.2982218564,
-0.4281981289,
-0.1595361829,
0.2162977606,
-0.2203807235,
-0.2502737939,
-0.1180728078,
-0.2273588777,
-0.1871369928,
-0.1366974115,
0.159518674,
0.0055198781,
0.3172376156,
-0.0935516208,
0.116638653,
-0.2562336028,
-0.6462205648,
0.1233225241,
0.211524725,
-0.1426579505,
0.0580332801,
0.199438706,
-0.2637610734,
0.1976294667,
0.7228574753,
0.251935631,
0.0261539593,
-0.0786248147,
0.0529664159,
0.0839555711,
0.0876961946,
-0.0001555171,
0.2521165907,
0.2659593225,
-0.6128063202,
0.1696701646,
-0.0114009343,
-0.1481028795,
0.4118888974,
-0.2929541171,
0.3268875182,
-0.3496899903,
0.4951539636,
-0.0461825393,
0.0140399979,
0.0405662954,
-0.1425221264,
-0.5717719197,
0.2246555239,
-0.0200582575,
-0.0718958452,
0.0829664543,
-0.3604297638,
0.0544082522,
-0.0736919343,
0.4507358074,
0.2547646463,
0.130871594,
-0.3133819997,
-0.0882292613,
-0.6293573976,
0.0641284436,
0.2504543364,
0.3122891784,
-0.0201937258,
0.2240371257,
0.0060549309,
0.1538951844,
0.2459769994,
-0.0620285831,
-0.0188191831,
-0.0547914654,
-0.6547976732,
0.2398652285,
-0.1182164699,
-0.0279669166,
0.1832563728,
0.1730832756,
0.0813004151,
-0.2520494163,
-0.0084852492,
0.2440533638,
-0.06250678,
-0.0889038518,
0.2176326513,
0.4322578907,
0.121643886,
0.0933315977,
0.0375455767,
-0.0467438027,
-0.2785559893,
-0.1413877755,
-0.193582207,
-0.0083426535,
-0.1165121347,
0.4362840652,
-0.3418883681,
-0.2784528732,
-0.3898363709,
0.2074488997,
0.0935019627,
-0.0974027216,
-0.1647611856,
0.40626508,
-0.0399050601,
-0.0485422537,
0.2773155272,
0.3931527734,
-0.0078774774,
0.3691283464,
-0.4815249443,
-0.2000588328,
0.7874456048,
-0.5091012716,
0.1622094959,
-0.2533198595,
0.3058441877,
0.4537868798,
-0.339070648,
-0.6162968874,
0.0236150678,
0.1255337298,
0.0467103943,
-0.1886404306,
0.2845036387,
-0.4269396961,
-0.1252789795,
-0.0973920152,
0.2794098854,
0.0648683831,
0.0003609281,
-0.0046301759,
-0.210304305
]
|
https://github.com/huggingface/datasets/issues/1942 | [experiment] missing default_experiment-1-0.arrow | Right, to clarify, I meant it'd be good to have it sorted on the library side and not requiring the user to figure it out. This is too complex and error-prone and if not coded correctly the bug will be intermittent which is even worse.
Oh I guess I wasn't clear in my message - in no way I'm proposing that we use this workaround code - I was just showing what I had to do to make it work.
We are on the same page.
> The changes you are proposing Stas are making the code less readable and also concatenate all the predictions and labels number_of_processes times I believe, which is not going to make the metric computation any faster.
And yes, this is another problem that my workaround introduces. Thank you for pointing it out, @sgugger
| the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you. | 139 | [experiment] missing default_experiment-1-0.arrow
the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you.
Right, to clarify, I meant it'd be good to have it sorted on the library side and not requiring the user to figure it out. This is too complex and error-prone and if not coded correctly the bug will be intermittent which is even worse.
Oh I guess I wasn't clear in my message - in no way I'm proposing that we use this workaround code - I was just showing what I had to do to make it work.
We are on the same page.
> The changes you are proposing Stas are making the code less readable and also concatenate all the predictions and labels number_of_processes times I believe, which is not going to make the metric computation any faster.
And yes, this is another problem that my workaround introduces. Thank you for pointing it out, @sgugger
| [
0.0017204055,
0.0483337082,
0.0741656646,
0.051863201,
0.0032178431,
-0.089067474,
0.2733487487,
0.3028242886,
0.2559508979,
0.1578379422,
0.0953508914,
0.1828420609,
-0.3078089058,
0.1192957833,
0.0508196466,
-0.0088097928,
-0.0289926771,
-0.1110829934,
-0.2872489691,
-0.054637678,
-0.2833440602,
0.5747833252,
-0.193736732,
0.0275072381,
-0.4175575674,
0.180084601,
-0.0967396349,
0.0959718227,
-0.0746752992,
-0.6333745122,
0.3431344926,
0.1919607967,
0.0437811874,
0.4339025319,
-0.00011918,
-0.1534664929,
0.2158040255,
-0.1456780136,
-0.0593992695,
-0.1108042374,
0.0721558854,
-0.3260094523,
0.4729872048,
-0.1469025761,
-0.0135607375,
-0.0872116089,
0.0065101404,
-0.4241973758,
0.3669989705,
0.0343662649,
0.1324311197,
0.2945925295,
-0.0216745734,
-0.292167902,
0.0711685494,
-0.1481085271,
0.0353576727,
0.3607212901,
-0.1339004934,
0.0896663368,
-0.2697996795,
0.2661952972,
0.2188456357,
-0.1134708077,
0.3259406984,
0.0134763364,
-0.0287554692,
-0.0886272714,
-0.1024972126,
0.2506767511,
0.2891424298,
-0.1953324229,
-0.2615005076,
0.0619684048,
-0.2091757357,
-0.3798714578,
0.1182646751,
-0.1241422743,
-0.0386009887,
0.0183158014,
0.066357404,
-0.1046713442,
-0.0890833288,
-0.0081160897,
-0.0986793563,
0.0158467311,
-0.1301836818,
0.046752464,
0.0187202878,
0.1890256107,
-0.3581736684,
0.4645083249,
0.0015241777,
0.1163826883,
-0.4526608586,
0.0794150755,
0.2340443283,
0.0034455371,
0.1275370419,
0.4340141416,
0.0486904979,
0.0966067836,
0.4923918247,
0.0736071765,
-0.1517193615,
0.545024693,
0.136821866,
-0.0942572281,
0.2153580189,
0.2788959742,
-0.0592240132,
-0.3349408507,
0.2765871882,
-0.2964961231,
0.3546168804,
0.1382147819,
0.0487447269,
-0.5286619663,
0.0650701374,
0.1770536751,
-0.1106245667,
-0.2184836417,
0.1539463997,
0.3556597531,
0.0642175525,
0.0728145018,
0.3231692016,
0.2419794053,
-0.2418756783,
0.0730487183,
-0.3921151459,
-0.0962858573,
-0.1561840177,
0.3900672495,
0.042701859,
-0.0673555508,
0.5413695574,
-0.2072089612,
0.3952535391,
-0.0542631596,
0.2074732631,
0.2733634412,
0.0856184512,
0.2450956106,
-0.0971159041,
0.110667862,
0.2532864213,
0.0348395444,
-0.2827268243,
-0.3384747207,
-0.1631170213,
-0.5935840011,
0.0358847342,
0.0273312181,
-0.4786866009,
0.172316134,
0.321629405,
0.3057263792,
-0.1627594382,
-0.1374481469,
0.1271646917,
0.0738493875,
-0.107867226,
-0.0605450682,
0.5470597148,
0.6318545341,
-0.3070906997,
-0.3632519245,
0.0832105428,
-0.0528886877,
0.0849455446,
0.1514369547,
0.0182660017,
0.1231782064,
-0.2710850537,
0.0660812184,
0.3751958609,
-0.2643638551,
-0.4211471379,
-0.0955257267,
-0.3790400326,
-0.1714234501,
0.1483896822,
0.0196402073,
0.2099821866,
0.1557331532,
0.0462167598,
-0.2413901389,
0.2183356881,
-0.2098486722,
-0.4395234585,
-0.3486972153,
0.0186813958,
-0.0433582105,
0.2190102488,
0.0419360921,
0.0363062136,
0.1706876606,
0.1256621182,
0.066039823,
0.179849416,
0.1231298745,
0.3237703741,
-0.0355295241,
0.2978155613,
0.044441577,
-0.2541937232,
0.1762871742,
-0.3174493909,
0.213625297,
0.1256955266,
-0.1005329937,
-0.2092878968,
-0.1439248621,
-0.0070457961,
-0.4147660732,
0.0263380595,
-0.0470578633,
0.2701241076,
0.1364538372,
-0.1191123724,
0.1284609288,
0.1072650552,
0.2114131302,
-0.4049315453,
0.0130384592,
-0.0703825876,
-0.3191621602,
0.0298932903,
0.1800132841,
0.0329501107,
0.1019102484,
-0.1698641777,
0.3908297718,
-0.1540257484,
0.2272180021,
0.3048530817,
0.2851656675,
-0.1629731357,
-0.3259808719,
0.0220397748,
0.110529393,
-0.0162727293,
0.0648802593,
-0.2022493482,
0.4049360156,
0.0456024036,
0.1391701698,
-0.2539227605,
0.2988233566,
-0.0855140686,
-0.0431872904,
-0.5963208079,
-0.0990096554,
0.3305993676,
-0.3289655447,
0.2105223686,
-0.1463252753,
-0.0314471498,
0.0823652521,
0.2473680377,
0.1233502552,
0.0772459656,
0.0978807658,
-0.1947133243,
-0.0856294036,
0.082913816,
-0.1318767071,
0.3044791818,
0.2182019055,
0.160731107,
0.108042486,
0.1258928031,
-0.1936117411,
0.1297410429,
0.0885623842,
-0.1993185282,
-0.0519844443,
0.2548466921,
0.1539466381,
-0.2797269523,
-0.0694241077,
-0.4146267176,
0.028454626,
-0.3391499817,
0.093310833,
-0.1681763679,
0.2760874331,
0.0427148566,
0.0185737442,
-0.2692074478,
-0.2986363471,
0.2447027564,
-0.2117598206,
-0.1059978604,
0.3040297031,
-0.327945739,
0.6078709364,
0.0554263592,
-0.1463518441,
-0.2999765277,
-0.0674370825,
-0.046668902,
-0.1146392524,
-0.2106841952,
0.2572808266,
0.2162182182,
-0.1139945909,
0.1512453705,
-0.0240463261,
-0.498768121,
0.0363868773,
0.0080176704,
0.6357992887,
0.3888978958,
0.0157713965,
-0.2617827058,
0.0316110365,
0.3991932273,
-0.4438588619,
-0.2224483341,
0.0916181579,
-0.0147887189,
0.2465288639,
-0.3239531517,
-0.4238111079,
0.1243079454,
-0.4012078643,
0.7106447816,
-0.0169693138,
0.0168242604,
-0.2196319401,
-0.0416192971,
0.2159824371,
-0.2111340165,
0.3168079555,
-0.3373787105,
-0.684572041,
0.4224869609,
-0.1047697738,
-0.2446504682,
-0.0255972967,
0.0576749817,
0.3486339748,
-0.1622273177,
-0.5894122124,
-0.4763512611,
-0.0748246685,
0.199990809,
-0.0194937997,
0.0612850115,
0.5578107834,
-0.0331991576,
-0.0518378057,
-0.0575104356,
-0.301984936,
0.615321219,
-0.2054655403,
0.04104257,
-0.007632514,
0.4433332384,
0.1814219356,
0.8166951537,
0.5588747263,
0.2393332571,
0.2656945884,
0.143451333,
0.3056058288,
-0.1414478868,
-0.0946218967,
0.318579644,
0.1920529753,
-0.0673524067,
0.1947142631,
0.1703395247,
0.1952800453,
-0.1114069,
0.0084028188,
-0.2526724637,
-0.3594485819,
-0.0285916962,
-0.0122554069,
0.1676211357,
-0.0411499403,
0.0335736573,
-0.2244507223,
-0.0431747846,
0.4540454745,
0.3833142221,
0.2945558131,
0.2433977872,
-0.1909155101,
0.1245272234,
-0.7305417061,
0.3448735178,
-0.4099698663,
-0.0014103298,
-0.1546725929,
-0.3290135562,
0.1191455051,
-0.059726458,
0.546821177,
0.2264778465,
-0.1941046119,
0.0241148211,
-0.2036363482,
-0.4357719123,
-0.0704815313,
-0.059580978,
-0.1430880725,
0.2688422799,
0.3386007845,
-0.4449521303,
-0.2397600561,
-0.1034352854,
-0.0312647074,
-0.2191043645,
-0.3313042521,
-0.2937083542,
-0.0996050164,
-0.2220920622,
0.2061283588,
-0.1314235926,
0.0842836648,
-0.0277878605,
0.0280266572,
-0.0842382461,
-0.1578320414,
0.0256603304,
0.1693504751,
0.3800824881,
-0.2912944853,
0.3020867407,
0.5443146229,
-0.0482205264,
0.0338874236,
0.3722674549,
0.0880227014,
-0.0148374988,
0.2595537007,
-0.0796130896,
0.318983078,
0.5834524035,
-0.3298604786,
0.1268919259,
-0.0379149616,
0.4513750374,
-0.2243208587,
-0.1023805439,
0.2226599008,
-0.0440627076,
0.395096451,
0.1574691832,
0.2453728765,
0.2686670125,
-0.2394674122,
0.1392651796,
0.0856610835,
-0.1921716034,
0.3677601814,
0.0769230202,
0.9748386741,
0.1425094604,
0.1349177659,
0.1659116745,
0.0843519047,
0.4501754344,
0.1098285094,
0.0794717446,
-0.2773194909,
-0.2535172403,
0.0752463341,
-0.1201171875,
-0.1260691434,
-0.3618938923,
-0.3251042962,
0.4081982076,
-0.2714812458,
-0.1056300774,
-0.2290644795,
-0.1442239881,
-0.2199870199,
-0.2697546482,
0.0447140113,
0.0453385301,
0.0907438472,
0.4384632111,
-0.1836979687,
-0.1840984523,
-0.2652371824,
-0.3652842939,
-0.128960669,
-0.0673228651,
-0.1766268015,
-0.1138672084,
0.2795241177,
0.0044976515,
-0.0696090907,
0.194792971,
0.3574464023,
0.1417983174,
-0.3306592405,
-0.0981991142,
0.0538100973,
0.1065853313,
0.1469285786,
0.0098673692,
0.2544250488,
-0.1431812495,
-0.0182386935,
-0.0244801063,
-0.1513184458,
0.0438991636,
0.0058427523,
-0.2144088745,
-0.0606864542,
-0.101869747,
0.098254174,
0.107100606,
0.011233151,
-0.2338056415,
0.110572122,
0.3235707283,
-0.1865551472,
0.1633544862,
0.2400681973,
-0.2253583223,
-0.0512892231,
0.4643435776,
-0.1365621835,
-0.0627512485,
0.4527921975,
0.042135451,
-0.1334979087,
-0.0853752643,
-0.0759428069,
0.3869661689,
-0.8485307693,
0.1576581597,
-0.0338790566,
-0.0515601523,
0.0432766974,
0.2604059279,
0.340882808,
-0.1588792056,
-0.082282491,
-0.2311332673,
-0.404238373,
0.381724745,
-0.305251658,
0.230650574,
-0.5099554062,
0.1902866066,
-0.2812500298,
0.1481529176,
-0.3190319836,
0.0882175863,
-0.220517382,
-0.1557302773,
-0.1763938665,
-0.0018250151,
0.3478408158,
0.0095738582,
-0.0726350248,
0.2380474061,
-0.370516181,
-0.0926235318,
-0.2068836987,
0.1353428513,
-0.1777231991,
0.0089448411,
0.0565367527,
0.227737844,
-0.1598330885,
-0.141060099,
0.0938877836,
0.1851565242,
-0.0096971421,
0.0880964175,
0.1260210723,
0.07567285,
-0.0135949971,
0.1977688968,
0.2378553003,
-0.1603399962,
-0.2263823301,
0.1338216662,
-0.4602912664,
-0.0737575814,
0.3484000564,
0.2329486161,
-0.1067645401,
0.246101737,
-0.0235695969,
0.0950313732,
-0.4482334852,
0.0451122522,
0.4445842803,
0.0489914268,
-0.0940123349,
-0.0108754793,
-0.0413266383,
0.0979491696,
-0.1402584463,
0.3291452527,
-0.0622718632,
0.3363083303,
0.1206547543,
0.276887387,
0.211622566,
0.0139382966,
0.1684415489,
0.2232993245,
-0.0547309071,
-0.091475144,
0.3676174879,
-0.1182743087,
0.0249971133,
0.3289466798,
0.5605509281,
0.0763844624,
0.1496206075,
-0.1066402942,
-0.0672026649,
0.1484336406,
-0.0661066771,
0.3817593455,
-0.2465206683,
-0.0910573155,
-0.1572406143,
0.1240019947,
0.0061646113,
-0.1901740283,
-0.3315696716,
0.6917665005,
-0.1093515754,
-0.2469646037,
-0.1880481541,
0.2186515629,
-0.1606263071,
-0.1849397123,
-0.0838894546,
-0.2105692923,
-0.2191711068,
-0.15095447,
0.1695629954,
0.0185523536,
0.1450959444,
-0.0328529142,
0.1160524637,
-0.1858572513,
-0.5609817505,
0.1418210119,
0.2941440344,
-0.1176032349,
0.0181689337,
0.2244017869,
-0.2845525444,
0.1979003847,
0.6598452926,
0.2762866318,
-0.0298538171,
-0.0884214565,
0.0559425279,
0.1904032677,
0.071514219,
-0.0744069591,
0.1938426197,
0.2686850429,
-0.6026167274,
0.1062570959,
0.0361008085,
-0.171439454,
0.4032694399,
-0.3423996866,
0.3624663055,
-0.244479239,
0.4835072458,
-0.1571029723,
0.1179203093,
0.002864416,
-0.1929029375,
-0.5489095449,
0.1697089523,
-0.1121425033,
-0.0506038889,
0.0383755974,
-0.3354281783,
0.0636245087,
-0.1064646766,
0.2458090484,
0.2641920447,
0.1838689893,
-0.2285052091,
-0.2114780396,
-0.5184432268,
0.1700243056,
0.2240598202,
0.4091751873,
0.0069526257,
0.2396065593,
-0.0212803427,
0.10178902,
0.2442371994,
0.0565917864,
-0.0967075825,
-0.0916240513,
-0.551802814,
0.1963034421,
-0.0378328897,
-0.1260571331,
0.1373005062,
0.1882485896,
-0.0334710218,
-0.2720346749,
0.0144161154,
0.1643150449,
-0.0944956318,
-0.0992467031,
0.1843334287,
0.4404117167,
0.2046841234,
0.0925298855,
-0.0192533135,
-0.0986652002,
-0.2265909463,
-0.1590010226,
-0.1725437492,
-0.1005910859,
-0.2516169548,
0.4177378714,
-0.2840111554,
-0.4044749141,
-0.3830953836,
0.2652685344,
0.0418740362,
-0.0697608963,
-0.2140797526,
0.42742154,
-0.1089173034,
-0.0424476638,
0.1821328104,
0.2948854864,
0.0220188145,
0.394222945,
-0.3259972632,
-0.2536974251,
0.796841681,
-0.4785505831,
0.2696473002,
-0.1940091997,
0.3755390644,
0.4057742953,
-0.3926135898,
-0.4662135243,
-0.0183523651,
0.0767981857,
0.1142916754,
-0.1075978652,
0.2290248424,
-0.4001033604,
-0.0505051613,
-0.0820425823,
0.3052809536,
0.168076247,
0.0183529481,
-0.0207120571,
-0.1692500561
]
|
https://github.com/huggingface/datasets/issues/1942 | [experiment] missing default_experiment-1-0.arrow | > The fact a datasets.Metric object cannot be used as a simple compute function in a multi-process environment is, in my opinion, a bug in datasets
Yes totally, this use case is supposed to be supported by `datasets`. And in this case there shouldn't be any collision between the metrics. I'm looking into it :)
My guess is that at one point the metric isn't using the right file name. It's supposed to use one with a unique uuid in order to avoid the collisions. | the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you. | 85 | [experiment] missing default_experiment-1-0.arrow
the original report was pretty bad and incomplete - my apologies!
Please see the complete version here: https://github.com/huggingface/datasets/issues/1942#issuecomment-786336481
------------
As mentioned here https://github.com/huggingface/datasets/issues/1939 metrics don't get cached, looking at my local `~/.cache/huggingface/metrics` - there are many `*.arrow.lock` files but zero metrics files.
w/o the network I get:
```
FileNotFoundError: [Errno 2] No such file or directory: '~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow
```
there is just `~/.cache/huggingface/metrics/sacrebleu/default/default_experiment-1-0.arrow.lock`
I did run the same `run_seq2seq.py` script on the instance with network and it worked just fine, but only the lock file was left behind.
this is with master.
Thank you.
> The fact a datasets.Metric object cannot be used as a simple compute function in a multi-process environment is, in my opinion, a bug in datasets
Yes totally, this use case is supposed to be supported by `datasets`. And in this case there shouldn't be any collision between the metrics. I'm looking into it :)
My guess is that at one point the metric isn't using the right file name. It's supposed to use one with a unique uuid in order to avoid the collisions. | [
-0.1335230172,
0.037119586,
0.0925974548,
0.2165249735,
0.1155614406,
0.0259056352,
0.2892279923,
0.2465748787,
0.2430669516,
0.1485800147,
0.0223329086,
0.1771803796,
-0.2399052382,
0.1134240329,
-0.0412851013,
-0.0090812147,
-0.032152351,
-0.0648061261,
-0.278406769,
-0.0532978289,
-0.371278137,
0.4944946468,
-0.2136930972,
0.057583116,
-0.4752726257,
0.0689632222,
-0.1987954378,
0.1876266152,
-0.120708406,
-0.4874229431,
0.3815603256,
0.1813317984,
0.0492469557,
0.6484947801,
-0.0001204537,
-0.1653452069,
0.2496982962,
-0.0945592299,
-0.1752889454,
-0.2627052367,
-0.0832368433,
-0.3161790371,
0.4207960367,
-0.212736845,
0.144111082,
-0.095614709,
0.03253977,
-0.4734925926,
0.3829444051,
0.0713168159,
0.1092244759,
0.4045967162,
-0.029013617,
-0.3105269969,
-0.0231044386,
-0.0660715327,
0.0089851152,
0.3649278283,
-0.1367455274,
0.0707328022,
-0.2014991939,
0.1797534227,
0.1654919535,
0.0047447402,
0.2488135993,
0.0069307634,
-0.0829744935,
-0.1220627725,
0.012220649,
0.3364714384,
0.2785384357,
-0.2549473047,
-0.3165335953,
0.0869155303,
-0.214629069,
-0.4481870234,
0.1836498678,
-0.1002554819,
0.0678672716,
0.0524203777,
-0.0563673787,
-0.1756006926,
-0.0516794175,
0.056594979,
-0.1596508175,
-0.1350141317,
-0.255456984,
0.1798074543,
0.0026670306,
0.1714438498,
-0.3266620934,
0.2590613663,
0.0486182906,
0.1027816162,
-0.5279275775,
0.2013272494,
0.1730943471,
-0.0289440211,
0.107283026,
0.4302812517,
-0.0310639162,
0.1589918584,
0.402990669,
0.1944816411,
-0.0551081151,
0.4064206779,
0.1782257855,
0.0014899902,
0.2801543772,
0.1749693751,
-0.2232089937,
-0.3422785699,
0.1502290219,
-0.3470237553,
0.3246974349,
0.0795397237,
0.1799818277,
-0.3473768532,
-0.0161982086,
0.1810606122,
-0.1118373945,
-0.1612889022,
0.0996880978,
0.2822612822,
0.1866632849,
-0.0516055226,
0.2157450914,
0.4055099189,
-0.1794903576,
0.0225589741,
-0.420535773,
-0.1213244125,
-0.1182070747,
0.4025938511,
0.0458072498,
-0.1762451828,
0.559706986,
-0.1764398366,
0.3955852389,
-0.14909935,
0.3421091437,
0.1321390569,
0.0209955107,
0.3017514646,
0.0507128499,
0.245919466,
0.270545274,
-0.003589642,
-0.102076225,
-0.3254131973,
-0.1897502393,
-0.5088412762,
0.0911111906,
-0.0049070651,
-0.4515235126,
0.2914337516,
0.2894194126,
0.2298966646,
-0.0728460103,
-0.2428895533,
0.0437055044,
0.0391707867,
-0.2443351597,
-0.1741627455,
0.5107160807,
0.6710849404,
-0.3306003213,
-0.346932739,
-0.0772820264,
-0.0398802422,
-0.0461153612,
0.2499622405,
-0.0406613164,
0.2462099642,
-0.282666266,
0.1334007978,
0.2764790356,
-0.3176392019,
-0.4375066161,
-0.0121186571,
-0.3603681624,
-0.1362519562,
0.0509689562,
-0.0041754488,
0.1422253698,
0.1615347266,
0.057849206,
-0.1849366575,
0.1697219312,
-0.3091488779,
-0.3021735549,
-0.2162058055,
0.0122870775,
-0.0623264797,
0.3494413495,
0.0934796035,
0.0866660997,
0.0533549413,
0.0463505276,
0.0644138008,
0.2311803699,
0.2167262137,
0.2976897657,
-0.0360913649,
0.3019514084,
-0.0816926956,
-0.2597104311,
0.3623228669,
-0.1630402058,
0.0962194875,
0.1023656204,
0.0269288663,
-0.1756435633,
-0.089235656,
-0.1704858691,
-0.4365578294,
0.0410098135,
-0.0181951616,
0.2263959199,
0.1631457657,
-0.1785070598,
0.2397719324,
0.1039648727,
0.1861557364,
-0.4449471533,
0.0735414252,
-0.0304206889,
-0.2937312424,
-0.0068507828,
0.1123707667,
0.0569213107,
0.0660692081,
-0.1975279748,
0.4633434415,
-0.0460407063,
0.2614343762,
0.430595845,
0.1458353251,
-0.1093312949,
-0.3408474922,
-0.0262469202,
-0.0013616382,
0.0535815619,
-0.0572734848,
-0.2175346762,
0.3621326983,
0.0230481196,
0.1479340941,
-0.246667549,
0.3745265603,
-0.0810130164,
-0.0561904721,
-0.5400526524,
-0.0346521735,
0.3048753738,
-0.2932925522,
0.3406457603,
-0.1490431428,
-0.0125546772,
-0.0973654911,
0.2400291711,
0.1414795667,
0.1339054406,
0.0312437806,
-0.3177609146,
-0.02832596,
0.0000273273,
-0.0193968937,
0.5301392078,
0.1484722346,
0.2678799629,
0.1348844171,
0.0870618448,
-0.1628808528,
0.0027406975,
0.0954414606,
0.0057383319,
0.0730258375,
0.2060079873,
0.1222143397,
-0.297286272,
-0.0545393154,
-0.2019446939,
0.0336646587,
-0.3863704205,
0.1017448008,
-0.2474816591,
0.4286674261,
0.1342418939,
0.1097209454,
-0.2200014889,
-0.2977716029,
0.1170778275,
-0.1271333843,
-0.0012804397,
0.3124437332,
-0.1825161725,
0.5101054907,
0.1200421304,
-0.1082286015,
-0.2282786667,
-0.1558557004,
-0.0329200104,
-0.1611798555,
-0.1028817743,
0.2642018199,
0.1315705925,
-0.1125557795,
0.1509695649,
-0.2350514978,
-0.4278851748,
0.0632875785,
0.0025527009,
0.7433798313,
0.4111455381,
-0.0036347811,
-0.279982239,
0.1605408639,
0.4311721027,
-0.4666098356,
-0.2351148576,
0.1392443627,
-0.0111169191,
0.0810800716,
-0.2477897704,
-0.3338741958,
0.067699194,
-0.3375908732,
0.6766371131,
-0.0679348037,
-0.0718750954,
-0.2298681885,
-0.0328821912,
0.2590989769,
-0.2438745797,
0.2232504934,
-0.3715209663,
-0.6017814279,
0.3536575139,
-0.1585827917,
-0.3658097684,
-0.0293398667,
0.0894151926,
0.4120743871,
0.0269325916,
-0.5248668194,
-0.4424892068,
-0.0557457916,
0.2325976044,
0.0255856533,
0.092356123,
0.4470263124,
-0.1033085361,
0.013612926,
-0.2129088789,
-0.3032338917,
0.588570714,
-0.1253107041,
0.0313163586,
-0.0246887356,
0.4544478357,
0.1785296947,
0.819362402,
0.573984921,
0.2213238329,
0.2435997128,
0.0272404291,
0.4192951024,
0.0100754704,
-0.2407456338,
0.3913175762,
0.0835299566,
-0.1487620473,
0.1663926095,
0.1160773784,
0.1952003986,
-0.1400609314,
0.0190408211,
-0.3352613449,
-0.2709818184,
-0.139850989,
-0.063785471,
0.2285215557,
0.1551153511,
0.0282765068,
-0.25396052,
0.0925768167,
0.3488355875,
0.3272808492,
0.1989056319,
0.1866466999,
-0.248255983,
0.0456900299,
-0.5986586213,
0.3302705288,
-0.4330096245,
0.1360399276,
-0.1070088744,
-0.1760904938,
0.1209392399,
-0.0360522196,
0.6881155968,
-0.0618486069,
-0.2268878669,
-0.0087779928,
-0.210758999,
-0.5308989286,
0.0313062593,
-0.1429649144,
0.0433674417,
0.2762993872,
0.4072861969,
-0.4166148901,
-0.1702870727,
-0.174085021,
0.023440307,
-0.2182138711,
-0.3281385899,
-0.3009985089,
-0.0614094734,
-0.2629105747,
0.0574810989,
-0.2308471352,
0.0075311535,
-0.0831387341,
0.0304851215,
-0.2600258291,
0.0171637796,
0.0119883474,
0.1035653502,
0.325992465,
-0.3720788062,
0.2957857847,
0.5614342093,
-0.0697109699,
0.1372146159,
0.4020886719,
0.0194239877,
-0.1499095112,
0.3707314134,
-0.0855319202,
0.2840799093,
0.4530299902,
-0.3448779583,
-0.0066802292,
-0.0185193531,
0.4108576179,
-0.1840827465,
-0.0980241001,
0.3913737833,
0.0029593189,
0.2447969317,
0.1449104846,
0.2343635708,
0.3837972879,
-0.1564327627,
0.2894921899,
0.0861309171,
-0.1000660136,
0.251170218,
0.0149510531,
0.8027763963,
0.0547764376,
0.0158253144,
0.1936586648,
0.0883167461,
0.5205698609,
0.1113065407,
-0.019903684,
-0.245632425,
-0.3328893483,
0.0005451217,
-0.1819362789,
0.0331190526,
-0.2277822345,
-0.2538830936,
0.3600266278,
-0.1687325239,
-0.13008973,
-0.1787786484,
-0.0495321006,
-0.2385252416,
-0.1991712153,
0.135170877,
0.0546529777,
0.1097514257,
0.4981808662,
-0.1706254184,
-0.1447526515,
-0.2722233236,
-0.3178368211,
-0.2482750118,
-0.0900003538,
-0.197752893,
-0.0111122355,
0.2499721497,
-0.0639045537,
-0.0252632722,
0.267781049,
0.5074552894,
0.0472656302,
-0.3525553644,
0.0034762048,
0.0130433142,
0.1437120289,
0.1161225066,
-0.1211204603,
0.2373107672,
-0.0689930618,
-0.092697002,
-0.0657149777,
-0.1648802906,
0.1208203435,
0.1018049493,
-0.1147972196,
-0.2134728581,
-0.0577049777,
0.0344789289,
0.0207515601,
0.0414714925,
-0.373603344,
0.0758248419,
0.272246927,
-0.146555528,
0.1722985506,
0.1113969237,
-0.2608965635,
-0.0141501669,
0.5147312284,
-0.2373294383,
-0.0303424578,
0.4104908109,
0.1308339983,
-0.028320238,
-0.1000232548,
0.0894253626,
0.4136508107,
-0.7102575898,
0.1728535444,
-0.0983265862,
0.1189660281,
0.0743288547,
0.1602975428,
0.2923332453,
-0.2233009487,
-0.0529580042,
-0.3134146929,
-0.3251955509,
0.2963857651,
-0.3277485669,
0.108809717,
-0.3996322453,
0.1098280326,
-0.1114409491,
0.2352113873,
-0.2688809633,
0.2411137372,
-0.2456685454,
-0.1734370589,
0.0200261474,
0.0298225507,
0.3511941433,
-0.0595264323,
-0.0846803412,
0.2033031881,
-0.357853353,
-0.0760750771,
-0.2357936651,
0.1430659294,
-0.0966830477,
0.0890797228,
0.0271420293,
0.173092261,
-0.1648471206,
-0.1966083199,
0.0914932862,
0.2636885941,
-0.0502027795,
0.0855075493,
0.1101144925,
0.1596432626,
-0.0471669659,
0.1796096712,
0.1540254951,
-0.1877580434,
-0.1319305152,
0.1650542468,
-0.2592051625,
-0.0534540005,
0.2167652696,
0.0128263496,
-0.1290295124,
0.1538246572,
0.069635801,
-0.0815031901,
-0.3034446537,
-0.0409244746,
0.586925149,
0.2290361822,
-0.1482737064,
0.0973779112,
-0.0870539919,
0.0747680143,
-0.1308402568,
0.2859938145,
0.0804746747,
0.2450647354,
0.1103253737,
0.1948862821,
0.1472148299,
0.0447328202,
-0.0332639292,
0.1988370866,
-0.0040261298,
-0.2192635238,
0.3405557871,
-0.1342509538,
0.0446110889,
0.3951192796,
0.5971512794,
0.1730560958,
0.1452832222,
0.1526365578,
-0.0955006182,
0.2259515375,
-0.1051746681,
0.3171735108,
-0.0828344673,
-0.1924598366,
-0.0912111178,
0.0132811284,
-0.0581705719,
-0.241695419,
-0.4781820476,
0.7504737973,
-0.3104271889,
-0.2048029602,
-0.2497941405,
0.2282945961,
-0.1533306092,
-0.1966205686,
-0.1678119302,
-0.1948920637,
-0.1005984843,
-0.1622462869,
0.1900170445,
0.0440238416,
0.2180063277,
-0.0209908355,
0.1075952128,
-0.365196377,
-0.5040789843,
0.2400597185,
0.0598785132,
-0.1469106674,
-0.037853945,
0.2424748093,
-0.310100615,
0.1404376626,
0.6258375049,
0.3339036703,
0.0355102718,
-0.1565676928,
0.0957119912,
0.201008752,
0.0026190479,
-0.112362355,
0.1926768869,
0.1783116013,
-0.4704281986,
0.2172093093,
0.0601484179,
-0.1306459457,
0.2637721002,
-0.2489311397,
0.3911229968,
-0.3739433885,
0.4142301679,
-0.3403811157,
0.1366838515,
0.0856257752,
-0.1665983796,
-0.5777475238,
0.2351000756,
0.0391674712,
-0.0788598955,
0.1047067568,
-0.3096340299,
0.049504254,
-0.1320973933,
0.2266009748,
0.3706275225,
0.1260538697,
-0.3011863232,
-0.2492924482,
-0.714277029,
0.3186248243,
0.126267463,
0.303276062,
-0.0899854749,
0.3800608814,
-0.0494269766,
0.0609032549,
0.2452538162,
0.0120627889,
-0.0164224114,
0.0251139067,
-0.5366048217,
0.0548593327,
-0.2019568384,
-0.1890062839,
0.1295389086,
0.2296338975,
0.0115954755,
-0.2051220387,
-0.0056058294,
0.0943917632,
-0.0876887292,
-0.1065064445,
0.1045287848,
0.4748959243,
0.2246724367,
0.117373243,
-0.0440186635,
-0.0084057543,
-0.2767063379,
-0.1092073768,
-0.0843692645,
-0.0301335808,
-0.3517563045,
0.4323346317,
-0.350294888,
-0.3416680396,
-0.3375522792,
0.1549042612,
0.1130458564,
-0.1122545749,
-0.2595624328,
0.4067004919,
-0.1336853802,
0.0444317423,
0.2433537841,
0.3771455288,
-0.0471836142,
0.4603344798,
-0.2675010264,
-0.1776426733,
0.7530429363,
-0.4552136064,
0.1326743364,
-0.2201700509,
0.3438363969,
0.3547529578,
-0.4648526013,
-0.3702156842,
0.0004913535,
0.1647121608,
0.0972569883,
-0.2037418485,
0.1568376869,
-0.3975250125,
-0.0358708128,
-0.0410870202,
0.2923150063,
0.1856916845,
-0.0826070979,
0.1278858632,
-0.0314364247
]
|
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.