repo
stringclasses 358
values | pull_number
int64 6
67.9k
| instance_id
stringlengths 12
49
| issue_numbers
sequencelengths 1
7
| base_commit
stringlengths 40
40
| patch
stringlengths 87
101M
| test_patch
stringlengths 72
22.3M
| problem_statement
stringlengths 3
256k
| hints_text
stringlengths 0
545k
| created_at
stringlengths 20
20
| PASS_TO_PASS
sequencelengths 0
0
| FAIL_TO_PASS
sequencelengths 0
0
|
---|---|---|---|---|---|---|---|---|---|---|---|
pypi/warehouse | 2,051 | pypi__warehouse-2051 | [
"162"
] | fb3ec3f9eabed2b60565bcb0efac74f694ac65c8 | diff --git a/warehouse/config.py b/warehouse/config.py
--- a/warehouse/config.py
+++ b/warehouse/config.py
@@ -93,8 +93,11 @@ def activate_hook(request):
return True
-def template_view(config, name, route, template):
- config.add_route(name, route)
+def template_view(config, name, route, template, route_kw=None):
+ if route_kw is None:
+ route_kw = {}
+
+ config.add_route(name, route, **route_kw)
config.add_view(renderer=template, route_name=name)
diff --git a/warehouse/forklift/__init__.py b/warehouse/forklift/__init__.py
--- a/warehouse/forklift/__init__.py
+++ b/warehouse/forklift/__init__.py
@@ -45,3 +45,11 @@ def includeme(config):
"doc_upload",
domain=forklift,
)
+
+ if forklift:
+ config.add_template_view(
+ "forklift.index",
+ "/",
+ "upload.html",
+ route_kw={"domain": forklift},
+ )
diff --git a/warehouse/routes.py b/warehouse/routes.py
--- a/warehouse/routes.py
+++ b/warehouse/routes.py
@@ -21,6 +21,10 @@ def includeme(config):
# Simple Route for health checks.
config.add_route("health", "/_health/")
+ # Internal route to make it easier to force a particular status for
+ # debugging HTTPException templates.
+ config.add_route("force-status", "/_force-status/{status:[45]\d\d}/")
+
# Basic global routes
config.add_route("index", "/", domain=warehouse)
config.add_route("robots.txt", "/robots.txt", domain=warehouse)
diff --git a/warehouse/views.py b/warehouse/views.py
--- a/warehouse/views.py
+++ b/warehouse/views.py
@@ -14,8 +14,9 @@
from pyramid.httpexceptions import (
HTTPException, HTTPSeeOther, HTTPMovedPermanently, HTTPNotFound,
- HTTPBadRequest,
+ HTTPBadRequest, exception_response,
)
+from pyramid.renderers import render_to_response
from pyramid.view import (
notfound_view_config, forbidden_view_config, view_config,
)
@@ -60,10 +61,41 @@
)
+# 403, 404, 410, 500,
+
+
@view_config(context=HTTPException)
@notfound_view_config(append_slash=HTTPMovedPermanently)
def httpexception_view(exc, request):
- return exc
+ # This special case exists for the easter egg that appears on the 404
+ # response page. We don't generally allow youtube embeds, but we make an
+ # except for this one.
+ if isinstance(exc, HTTPNotFound):
+ request.find_service(name="csp").merge({
+ "frame-src": ["https://www.youtube-nocookie.com"],
+ "script-src": ["https://www.youtube.com", "https://s.ytimg.com"],
+ })
+
+ try:
+ response = render_to_response(
+ "{}.html".format(exc.status_code),
+ {},
+ request=request,
+ )
+ except LookupError:
+ # We don't have a customized template for this error, so we'll just let
+ # the default happen instead.
+ return exc
+
+ # Copy over the important values from our HTTPException to our new response
+ # object.
+ response.status = exc.status
+ response.headers.extend(
+ (k, v) for k, v in exc.headers.items()
+ if k not in response.headers
+ )
+
+ return response
@forbidden_view_config()
@@ -79,8 +111,7 @@ def forbidden(exc, request, redirect_to="accounts.login"):
# If we've reached here, then the user is logged in and they are genuinely
# not allowed to access this page.
- # TODO: Style the forbidden page.
- return exc
+ return httpexception_view(exc, request)
@view_config(
@@ -311,3 +342,11 @@ def health(request):
# Nothing will actually check this, but it's a little nicer to have
# something to return besides an empty body.
return "OK"
+
+
+@view_config(route_name="force-status")
+def force_status(request):
+ try:
+ raise exception_response(int(request.matchdict["status"]))
+ except KeyError:
+ raise exception_response(404) from None
| diff --git a/tests/unit/forklift/test_init.py b/tests/unit/forklift/test_init.py
--- a/tests/unit/forklift/test_init.py
+++ b/tests/unit/forklift/test_init.py
@@ -26,6 +26,7 @@ def test_includeme(forklift):
get_settings=lambda: settings,
include=pretend.call_recorder(lambda n: None),
add_legacy_action_route=pretend.call_recorder(lambda *a, **k: None),
+ add_template_view=pretend.call_recorder(lambda *a, **kw: None),
)
includeme(config)
@@ -49,3 +50,14 @@ def test_includeme(forklift):
domain=forklift,
),
]
+ if forklift:
+ config.add_template_view.calls == [
+ pretend.call(
+ "forklift.index",
+ "/",
+ "upload.html",
+ route_kw={"domain": forklift},
+ ),
+ ]
+ else:
+ config.add_template_view.calls == []
diff --git a/tests/unit/test_config.py b/tests/unit/test_config.py
--- a/tests/unit/test_config.py
+++ b/tests/unit/test_config.py
@@ -97,15 +97,20 @@ def test_activate_hook(path, expected):
assert config.activate_hook(request) == expected
-def test_template_view():
[email protected]("route_kw", [None, {}, {"foo": "bar"}])
+def test_template_view(route_kw):
configobj = pretend.stub(
add_route=pretend.call_recorder(lambda *a, **kw: None),
add_view=pretend.call_recorder(lambda *a, **kw: None),
)
- config.template_view(configobj, "test", "/test/", "test.html")
+ config.template_view(configobj, "test", "/test/", "test.html",
+ route_kw=route_kw)
- assert configobj.add_route.calls == [pretend.call("test", "/test/")]
+ assert configobj.add_route.calls == [
+ pretend.call(
+ "test", "/test/", **({} if route_kw is None else route_kw)),
+ ]
assert configobj.add_view.calls == [
pretend.call(renderer="test.html", route_name="test"),
]
diff --git a/tests/unit/test_routes.py b/tests/unit/test_routes.py
--- a/tests/unit/test_routes.py
+++ b/tests/unit/test_routes.py
@@ -73,6 +73,7 @@ def add_policy(name, filename):
assert config.add_route.calls == [
pretend.call("health", "/_health/"),
+ pretend.call("force-status", "/_force-status/{status:[45]\d\d}/"),
pretend.call('index', '/', domain=warehouse),
pretend.call("robots.txt", "/robots.txt", domain=warehouse),
pretend.call("opensearch.xml", "/opensearch.xml", domain=warehouse),
diff --git a/tests/unit/test_views.py b/tests/unit/test_views.py
--- a/tests/unit/test_views.py
+++ b/tests/unit/test_views.py
@@ -24,7 +24,7 @@
from warehouse import views
from warehouse.views import (
SEARCH_BOOSTS, SEARCH_FIELDS, current_user_indicator, forbidden, health,
- httpexception_view, index, robotstxt, opensearchxml, search
+ httpexception_view, index, robotstxt, opensearchxml, search, force_status,
)
from ..common.db.accounts import UserFactory
@@ -34,18 +34,79 @@
)
-def test_httpexception_view():
- response = context = pretend.stub()
- request = pretend.stub()
- assert httpexception_view(context, request) is response
+class TestHTTPExceptionView:
+
+ def test_returns_context_when_no_template(self, pyramid_config):
+ pyramid_config.testing_add_renderer("non-existent.html")
+
+ response = context = pretend.stub(status_code=499)
+ request = pretend.stub()
+ assert httpexception_view(context, request) is response
+
+ @pytest.mark.parametrize("status_code", [403, 404, 410, 500])
+ def test_renders_template(self, pyramid_config, status_code):
+ renderer = pyramid_config.testing_add_renderer(
+ "{}.html".format(status_code))
+
+ context = pretend.stub(
+ status="{} My Cool Status".format(status_code),
+ status_code=status_code,
+ headers={},
+ )
+ request = pretend.stub()
+ response = httpexception_view(context, request)
+
+ assert response.status_code == status_code
+ assert response.status == "{} My Cool Status".format(status_code)
+ renderer.assert_()
+
+ @pytest.mark.parametrize("status_code", [403, 404, 410, 500])
+ def test_renders_template_with_headers(self, pyramid_config, status_code):
+ renderer = pyramid_config.testing_add_renderer(
+ "{}.html".format(status_code))
+
+ context = pretend.stub(
+ status="{} My Cool Status".format(status_code),
+ status_code=status_code,
+ headers={"Foo": "Bar"},
+ )
+ request = pretend.stub()
+ response = httpexception_view(context, request)
+
+ assert response.status_code == status_code
+ assert response.status == "{} My Cool Status".format(status_code)
+ assert response.headers["Foo"] == "Bar"
+ renderer.assert_()
+
+ def test_renders_404_with_csp(self, pyramid_config):
+ renderer = pyramid_config.testing_add_renderer("404.html")
+
+ csp = {}
+ services = {"csp": pretend.stub(merge=csp.update)}
+
+ context = HTTPNotFound()
+ request = pretend.stub(find_service=lambda name: services[name])
+ response = httpexception_view(context, request)
+
+ assert response.status_code == 404
+ assert response.status == "404 Not Found"
+ assert csp == {
+ "frame-src": ["https://www.youtube-nocookie.com"],
+ "script-src": ["https://www.youtube.com", "https://s.ytimg.com"],
+ }
+ renderer.assert_()
class TestForbiddenView:
- def test_logged_in_returns_exception(self):
- exc, request = pretend.stub(), pretend.stub(authenticated_userid=1)
+ def test_logged_in_returns_exception(self, pyramid_config):
+ renderer = pyramid_config.testing_add_renderer("403.html")
+
+ exc = pretend.stub(status_code=403, status="403 Forbidden", headers={})
+ request = pretend.stub(authenticated_userid=1)
resp = forbidden(exc, request)
- assert resp is exc
+ assert resp.status_code == 403
+ renderer.assert_()
def test_logged_out_redirects_login(self):
exc = pretend.stub()
@@ -450,3 +511,14 @@ def test_health():
assert health(request) == "OK"
assert request.db.execute.calls == [pretend.call("SELECT 1")]
+
+
+class TestForceStatus:
+
+ def test_valid(self):
+ with pytest.raises(HTTPBadRequest):
+ force_status(pretend.stub(matchdict={"status": "400"}))
+
+ def test_invalid(self):
+ with pytest.raises(HTTPNotFound):
+ force_status(pretend.stub(matchdict={"status": "599"}))
| Properly style the error pages
This includes (but is probably not limited too):
- [ ] 500
- [ ] 404
- [ ] 410 (Maybe? Not sure if we'll ever use it.)
- [ ] 403
| One thing that I think would be kind of cute to do, is on the 404 page, embed a link to https://www.youtube.com/watch?v=cWDdd5KKhts as an easter-egg referencing both the "unofficial" name of PyPI and the fact that the skit itself is about a cheeseshop that's missing any cheese... essentially a 404 ;)
Bonus points if we stick the real 404 content at the top, then lead into the embedded youtube with a "And now for something completely different".
| 2017-05-28T20:24:20Z | [] | [] |
pypi/warehouse | 2,054 | pypi__warehouse-2054 | [
"1912"
] | f2b4484e374f3de0acff717bef90d0522083336d | diff --git a/warehouse/config.py b/warehouse/config.py
--- a/warehouse/config.py
+++ b/warehouse/config.py
@@ -284,11 +284,14 @@ def configure(settings=None):
renderers.JSON(sort_keys=True, separators=(",", ":")),
)
+ # Configure retry support.
+ config.add_settings({"retry.attempts": 3})
+ config.include("pyramid_retry")
+
# Configure our transaction handling so that each request gets its own
# transaction handler and the lifetime of the transaction is tied to the
# lifetime of the request.
config.add_settings({
- "tm.attempts": 3,
"tm.manager_hook": lambda request: transaction.TransactionManager(),
"tm.activate_hook": activate_hook,
"tm.annotate_user": False,
diff --git a/warehouse/tasks.py b/warehouse/tasks.py
--- a/warehouse/tasks.py
+++ b/warehouse/tasks.py
@@ -21,6 +21,7 @@
import celery
import celery.backends.redis
import pyramid.scripting
+import pyramid_retry
import transaction
import venusian
@@ -56,7 +57,8 @@ def run(*args, **kwargs):
try:
return original_run(*args, **kwargs)
except BaseException as exc:
- if request.tm._retryable(exc.__class__, exc):
+ if (isinstance(exc, pyramid_retry.RetryableException)
+ or pyramid_retry.IRetryableError.providedBy(exc)):
raise obj.retry(exc=exc)
raise
| diff --git a/tests/unit/test_config.py b/tests/unit/test_config.py
--- a/tests/unit/test_config.py
+++ b/tests/unit/test_config.py
@@ -319,6 +319,7 @@ def __init__(self):
),
]
] + [
+ pretend.call("pyramid_retry"),
pretend.call("pyramid_tm"),
pretend.call("pyramid_services"),
pretend.call("pyramid_rpc.xmlrpc"),
@@ -365,8 +366,8 @@ def __init__(self):
]
assert configurator_obj.add_settings.calls == [
pretend.call({"jinja2.newstyle": True}),
+ pretend.call({"retry.attempts": 3}),
pretend.call({
- "tm.attempts": 3,
"tm.manager_hook": mock.ANY,
"tm.activate_hook": config.activate_hook,
"tm.annotate_user": False,
@@ -377,7 +378,7 @@ def __init__(self):
},
}),
]
- add_settings_dict = configurator_obj.add_settings.calls[1].args[0]
+ add_settings_dict = configurator_obj.add_settings.calls[2].args[0]
assert add_settings_dict["tm.manager_hook"](pretend.stub()) is \
transaction_manager
assert configurator_obj.add_tween.calls == [
diff --git a/tests/unit/test_tasks.py b/tests/unit/test_tasks.py
--- a/tests/unit/test_tasks.py
+++ b/tests/unit/test_tasks.py
@@ -18,6 +18,7 @@
from celery import Celery
from pyramid import scripting
+from pyramid_retry import RetryableException
from warehouse import tasks
from warehouse.config import Environment
@@ -240,7 +241,7 @@ def run(arg_, *, kwarg_=None):
assert request.tm.__exit__.calls == [pretend.call(None, None, None)]
def test_run_retries_failed_transaction(self):
- class RetryThisException(Exception):
+ class RetryThisException(RetryableException):
pass
class Retry(Exception):
@@ -259,7 +260,6 @@ def run():
tm=pretend.stub(
__enter__=pretend.call_recorder(lambda *a, **kw: None),
__exit__=pretend.call_recorder(lambda *a, **kw: None),
- _retryable=pretend.call_recorder(lambda *a, **kw: True),
),
)
@@ -273,9 +273,6 @@ def run():
assert request.tm.__exit__.calls == [
pretend.call(Retry, mock.ANY, mock.ANY),
]
- assert request.tm._retryable.calls == [
- pretend.call(RetryThisException, mock.ANY),
- ]
def test_run_doesnt_retries_failed_transaction(self):
class DontRetryThisException(Exception):
@@ -294,7 +291,6 @@ def run():
tm=pretend.stub(
__enter__=pretend.call_recorder(lambda *a, **kw: None),
__exit__=pretend.call_recorder(lambda *a, **kw: None),
- _retryable=pretend.call_recorder(lambda *a, **kw: False),
),
)
@@ -308,9 +304,6 @@ def run():
assert request.tm.__exit__.calls == [
pretend.call(DontRetryThisException, mock.ANY, mock.ANY),
]
- assert request.tm._retryable.calls == [
- pretend.call(DontRetryThisException, mock.ANY),
- ]
def test_after_return_without_pyramid_env(self):
obj = tasks.WarehouseTask()
| Update pyramid-tm to 2.0
There's a new version of [pyramid-tm](https://pypi.python.org/pypi/pyramid-tm) available.
You are currently using **1.1.1**. I have updated it to **2.0**
These links might come in handy: <a href="http://pypi.python.org/pypi/pyramid_tm">PyPI</a> | <a href="https://pyup.io/changelogs/pyramid-tm/">Changelog</a> | <a href="http://docs.pylonsproject.org/projects/pyramid-tm/en/latest/">Homepage</a>
### Changelog
>
>### 2.0
>^^^^^^^^^^^^^^^^
>Major Features
>~~~~~~~~~~~~~~
>- The ``pyramid_tm`` tween has been moved **over** the ``EXCVIEW`` tween.
> This means the transaction is open during exception view execution.
> See https://github.com/Pylons/pyramid_tm/pull/55
>- Added a ``pyramid_tm.is_tm_active`` and a ``tm_active`` view predicate
> which may be useful in exception views that require access to the database.
> See https://github.com/Pylons/pyramid_tm/pull/60
>Backward Incompatibilities
>~~~~~~~~~~~~~~~~~~~~~~~~~~
>- The ``tm.attempts`` setting has been removed and retry support has been moved
> into a new package named ``pyramid_retry``. If you want retry support then
> please look at that library for more information about installing and
> enabling it. See https://github.com/Pylons/pyramid_tm/pull/55
>- The ``pyramid_tm`` tween has been moved **over** the ``EXCVIEW`` tween.
> If you have any hacks in your application that are opening a new transaction
> inside your exception views then it's likely you will want to remove them
> or re-evaluate when upgrading.
> See https://github.com/Pylons/pyramid_tm/pull/55
>- Drop support for Pyramid < 1.5.
>Minor Features
>~~~~~~~~~~~~~~
>- Support for Python 3.6.
*Got merge conflicts? Close this PR and delete the branch. I'll create a new PR for you.*
Happy merging! 🤖
| This probably needs to wait until Pyramid 1.9 is released so that we can switch to pyramid_retry to handle retry logic. | 2017-05-29T18:10:36Z | [] | [] |
pypi/warehouse | 2,473 | pypi__warehouse-2473 | [
"2471"
] | da5dc63136c4f351c2ac8d4e93878db7ea8caabe | diff --git a/warehouse/admin/views/projects.py b/warehouse/admin/views/projects.py
--- a/warehouse/admin/views/projects.py
+++ b/warehouse/admin/views/projects.py
@@ -24,6 +24,9 @@
from warehouse.accounts.models import User
from warehouse.packaging.models import Project, Release, Role, JournalEntry
from warehouse.utils.paginate import paginate_url_factory
+from warehouse.forklift.legacy import MAX_FILESIZE
+
+ONE_MB = 1024 * 1024 # bytes
@view_config(
@@ -101,7 +104,13 @@ def project_detail(project, request):
)
]
- return {"project": project, "maintainers": maintainers, "journal": journal}
+ return {
+ "project": project,
+ "maintainers": maintainers,
+ "journal": journal,
+ "ONE_MB": ONE_MB,
+ "MAX_FILESIZE": MAX_FILESIZE
+ }
@view_config(
@@ -218,18 +227,27 @@ def set_upload_limit(project, request):
# If the upload limit is an empty string or othrwise falsy, just set the
# limit to None, indicating the default limit.
if not upload_limit:
- project.upload_limit = None
+ upload_limit = None
else:
try:
- project.upload_limit = int(upload_limit)
+ upload_limit = int(upload_limit)
except ValueError:
raise HTTPBadRequest(
- f"Invalid value for upload_limit: {upload_limit}, "
+ f"Invalid value for upload limit: {upload_limit}, "
f"must be integer or empty string.")
+ # The form is in MB, but the database field is in bytes.
+ upload_limit *= ONE_MB
+
+ if upload_limit < MAX_FILESIZE:
+ raise HTTPBadRequest(
+ f"Upload limit can not be less than the default limit of "
+ f"{MAX_FILESIZE / ONE_MB}MB.")
+
+ project.upload_limit = upload_limit
+
request.session.flash(
- f"Successfully set the upload limit on {project.name!r} to "
- f"{project.upload_limit!r}",
+ f"Successfully set the upload limit on {project.name!r}.",
queue="success",
)
| diff --git a/tests/unit/admin/views/test_projects.py b/tests/unit/admin/views/test_projects.py
--- a/tests/unit/admin/views/test_projects.py
+++ b/tests/unit/admin/views/test_projects.py
@@ -354,21 +354,21 @@ def test_sets_limitwith_integer(self, db_request):
flash=pretend.call_recorder(lambda *a, **kw: None),
)
db_request.matchdict["project_name"] = project.normalized_name
- db_request.POST["upload_limit"] = "12345"
+ db_request.POST["upload_limit"] = "90"
views.set_upload_limit(project, db_request)
assert db_request.session.flash.calls == [
pretend.call(
- "Successfully set the upload limit on 'foo' to 12345",
+ "Successfully set the upload limit on 'foo'.",
queue="success"),
]
- assert project.upload_limit == 12345
+ assert project.upload_limit == 90 * views.ONE_MB
def test_sets_limit_with_none(self, db_request):
project = ProjectFactory.create(name="foo")
- project.upload_limit = 12345
+ project.upload_limit = 90 * views.ONE_MB
db_request.route_path = pretend.call_recorder(
lambda *a, **kw: "/admin/projects/")
@@ -381,13 +381,13 @@ def test_sets_limit_with_none(self, db_request):
assert db_request.session.flash.calls == [
pretend.call(
- "Successfully set the upload limit on 'foo' to None",
+ "Successfully set the upload limit on 'foo'.",
queue="success"),
]
assert project.upload_limit is None
- def test_sets_limit_with_bad_value(self, db_request):
+ def test_sets_limit_with_non_integer(self, db_request):
project = ProjectFactory.create(name="foo")
db_request.matchdict["project_name"] = project.normalized_name
@@ -395,3 +395,12 @@ def test_sets_limit_with_bad_value(self, db_request):
with pytest.raises(HTTPBadRequest):
views.set_upload_limit(project, db_request)
+
+ def test_sets_limit_with_less_than_minimum(self, db_request):
+ project = ProjectFactory.create(name="foo")
+
+ db_request.matchdict["project_name"] = project.normalized_name
+ db_request.POST["upload_limit"] = "20"
+
+ with pytest.raises(HTTPBadRequest):
+ views.set_upload_limit(project, db_request)
| Admin package upload size text control should use MB instead of bytes
Context: https://github.com/pypa/warehouse/pull/2470#issuecomment-334852617
Decision:
> The input in should be in MB and should just be converted to/from bytes in the backend.
Also:
> It also might be a good idea to flash an error and redirect back to the detail page if you try to set a limit less then the current default minimum.
Additionally:
> [The form field] would be a bit nicer as <input type="number" min={{ THE MINMUM SIZE }} ...> instead of as a text field. It might also make sense to include step="10" or something
| @dstufft I'm a PyPA member but not a member on this project so I can't assign myself. | 2017-10-06T21:39:21Z | [] | [] |
pypi/warehouse | 2,524 | pypi__warehouse-2524 | [
"2489"
] | 4419dcd714a7f29294e7f4ca03bb75a42ee40216 | diff --git a/warehouse/accounts/forms.py b/warehouse/accounts/forms.py
--- a/warehouse/accounts/forms.py
+++ b/warehouse/accounts/forms.py
@@ -29,6 +29,18 @@ class CredentialsMixin:
"a username with under 50 characters."
)
),
+ # the regexp below must match the CheckConstraint
+ # for the username field in accounts.models.User
+ wtforms.validators.Regexp(
+ r'^[a-zA-Z0-9][a-zA-Z0-9._-]*[a-zA-Z0-9]$',
+ message=(
+ "The username is invalid. Usernames "
+ "must be composed of letters, numbers, "
+ "dots, hyphens and underscores. And must "
+ "also start and finish with a letter or number. "
+ "Please choose a different username."
+ )
+ )
],
)
| diff --git a/tests/unit/accounts/test_forms.py b/tests/unit/accounts/test_forms.py
--- a/tests/unit/accounts/test_forms.py
+++ b/tests/unit/accounts/test_forms.py
@@ -311,6 +311,28 @@ def test_username_exists(self):
"Please choose a different username."
)
+ @pytest.mark.parametrize("username", ['_foo', 'bar_', 'foo^bar'])
+ def test_username_is_valid(self, username):
+ form = forms.RegistrationForm(
+ data={"username": username},
+ user_service=pretend.stub(
+ find_userid=pretend.call_recorder(lambda _: None),
+ ),
+ recaptcha_service=pretend.stub(
+ enabled=False,
+ verify_response=pretend.call_recorder(lambda _: None),
+ ),
+ )
+ assert not form.validate()
+ assert (
+ form.username.errors.pop() ==
+ "The username is invalid. Usernames "
+ "must be composed of letters, numbers, "
+ "dots, hyphens and underscores. And must "
+ "also start and finish with a letter or number. "
+ "Please choose a different username."
+ )
+
def test_password_strength(self):
cases = (
("foobar", False),
| Validate username format before attempting to flush it to the db
Validate a valid username prior to trying to save it to the database, using the same logic as is already in the database.
---
https://sentry.io/python-software-foundation/warehouse-production/issues/258353802/
```
IntegrityError: new row for relation "accounts_user" violates check constraint "accounts_user_valid_username"
File "sqlalchemy/engine/base.py", line 1182, in _execute_context
context)
File "sqlalchemy/engine/default.py", line 470, in do_execute
cursor.execute(statement, parameters)
IntegrityError: (psycopg2.IntegrityError) new row for relation "accounts_user" violates check constraint "accounts_user_valid_username"
(30 additional frame(s) were not displayed)
...
File "warehouse/raven.py", line 41, in raven_tween
return handler(request)
File "warehouse/cache/http.py", line 69, in conditional_http_tween
response = handler(request)
File "warehouse/cache/http.py", line 33, in wrapped
return view(context, request)
File "warehouse/accounts/views.py", line 226, in register
form.email.data
File "warehouse/accounts/services.py", line 152, in create_user
self.db.flush()
IntegrityError: (psycopg2.IntegrityError) new row for relation "accounts_user" violates check constraint "accounts_user_valid_username"
DETAIL: Failing row contains
```
| 2017-10-21T13:19:53Z | [] | [] |
|
pypi/warehouse | 2,574 | pypi__warehouse-2574 | [
"2217"
] | ef47a00b7d16c94244ab1991957aea9b90e12dd3 | diff --git a/warehouse/legacy/api/simple.py b/warehouse/legacy/api/simple.py
--- a/warehouse/legacy/api/simple.py
+++ b/warehouse/legacy/api/simple.py
@@ -10,6 +10,8 @@
# See the License for the specific language governing permissions and
# limitations under the License.
+
+from packaging.version import parse
from pyramid.httpexceptions import HTTPMovedPermanently
from pyramid.view import view_config
from sqlalchemy import func
@@ -73,7 +75,7 @@ def simple_detail(project, request):
request.response.headers["X-PyPI-Last-Serial"] = str(project.last_serial)
# Get all of the files for this project.
- files = (
+ files = sorted(
request.db.query(File)
.options(joinedload(File.release))
.filter(
@@ -84,8 +86,8 @@ def simple_detail(project, request):
.with_entities(Release.version)
)
)
- .order_by(File.filename)
- .all()
+ .all(),
+ key=lambda f: (parse(f.version), f.packagetype)
)
return {"project": project, "files": files}
| diff --git a/tests/unit/legacy/api/test_simple.py b/tests/unit/legacy/api/test_simple.py
--- a/tests/unit/legacy/api/test_simple.py
+++ b/tests/unit/legacy/api/test_simple.py
@@ -161,3 +161,64 @@ def test_with_files_with_serial(self, db_request):
"files": files,
}
assert db_request.response.headers["X-PyPI-Last-Serial"] == str(je.id)
+
+ def test_with_files_with_version_multi_digit(self, db_request):
+ project = ProjectFactory.create()
+ releases = [
+ ReleaseFactory.create(project=project)
+ for _ in range(3)
+ ]
+ release_versions = [
+ "0.3.0rc1", "0.3.0", "0.3.0-post0", "0.14.0", "4.2.0", "24.2.0"
+ ]
+
+ for release, version in zip(releases, release_versions):
+ release.version = version
+
+ tar_files = [
+ FileFactory.create(
+ release=r,
+ filename="{}-{}.tar.gz".format(project.name, r.version),
+ packagetype="sdist"
+ )
+ for r in releases
+ ]
+ wheel_files = [
+ FileFactory.create(
+ release=r,
+ filename="{}-{}.whl".format(project.name, r.version),
+ packagetype="bdist_wheel"
+ )
+ for r in releases
+ ]
+ egg_files = [
+ FileFactory.create(
+ release=r,
+ filename="{}-{}.egg".format(project.name, r.version),
+ packagetype="bdist_egg"
+ )
+ for r in releases
+ ]
+
+ files = []
+ for files_release in \
+ zip(egg_files, wheel_files, tar_files):
+ files += files_release
+
+ db_request.matchdict["name"] = project.normalized_name
+ user = UserFactory.create()
+ je = JournalEntryFactory.create(name=project.name, submitted_by=user)
+
+ # Make sure that we get any changes made since the JournalEntry was
+ # saved.
+ db_request.db.refresh(project)
+ import pprint
+ pprint.pprint(simple.simple_detail(project, db_request)['files'])
+ pprint.pprint(files)
+
+ assert simple.simple_detail(project, db_request) == {
+ "project": project,
+ "files": files,
+ }
+
+ assert db_request.response.headers["X-PyPI-Last-Serial"] == str(je.id)
| Improve sorting on simple page
I'd like to submit a patch for this but I have a few questions :)
First I'll describe what I'd like to do...
## sort by version number
See https://pypi.org/simple/pre-commit/
You'll notice that `0.10.0` erroneously sorts *before* `0.2.0` (I'd like to fix this)
## investigation
I've found the code which does this sorting [here](https://github.com/pypa/warehouse/blob/3bdfe5a89cc9a922ee97304c98384c24822a09ee/warehouse/legacy/api/simple.py#L76-L89)
This seems to just sort by filename, but by inspecting and viewing [this page](https://pypi.org/simple/pre-commit-mirror-maker/) I notice it seems to ignore `_` vs. `-` (which is good, that's what I want to continue to happen but I'm just not seeing it from the code!)
## other questions
The `File` objects which come back from the database contain a `.version` attribute that I'd like to use to participate in sorting, my main question is: **Can I depend on this version to be a valid [PEP440](https://www.python.org/dev/peps/pep-0440/) version and use something like `pkg_resources.parse_version`?**
I'd basically like to replicate something close to the sorting which @chriskuehl's [dumb-pypi](https://github.com/chriskuehl/dumb-pypi) does [here](https://github.com/chriskuehl/dumb-pypi/blob/fd0f93fc2e82cbd9bae41b3c60c5f006b2319c60/dumb_pypi/main.py#L77-L91).
Thanks in advance :)
---
**Good First Issue**: This issue is good for first time contributors. If there is not a corresponding pull request for this issue, it is up for grabs. For directions for getting set up, see our [Getting Started Guide](https://warehouse.pypa.io/development/getting-started/). If you are working on this issue and have questions, please feel free to ask them here, [`#pypa-dev` on Freenode](https://webchat.freenode.net/?channels=%23pypa-dev), or the [pypa-dev mailing list](https://groups.google.com/forum/#!forum/pypa-dev).
| It does just sort by filename, largely because the order doesn't *really* matter, as long as it's stable between page loads. That being said, I wouldn't turn down a PR that does this. The version attributes are not guaranteed to be PEP 440 compatible (some of them are old enough that they predate PEP 440). Ideally you're use ``packaging.version.parse_version`` which will handle that case fine.
@dstufft if it sorts by filename, I would expect the following sorting on this page: https://pypi.org/simple/pre-commit-mirror-maker/
```python
[
'pre-commit-mirror-maker-0.1.0.tar.gz',
'pre-commit-mirror-maker-0.1.1.tar.gz',
'pre-commit-mirror-maker-0.1.2.tar.gz',
'pre-commit-mirror-maker-0.2.0.tar.gz',
'pre-commit-mirror-maker-0.3.0.tar.gz',
'pre-commit-mirror-maker-0.3.1.tar.gz',
'pre-commit-mirror-maker-0.3.2.tar.gz',
'pre-commit-mirror-maker-0.4.0.tar.gz',
'pre_commit_mirror_maker-0.3.2-py2-none-any.whl',
'pre_commit_mirror_maker-0.4.0-py2-none-any.whl',
'pre_commit_mirror_maker-0.4.0-py3-none-any.whl',
]
```
but the wheel files are properly sorted alongside their source distributions -- does postgres normalize this?
I don't honestly recall the specifics of how it sorts other than it was by filename.
Awesome, thanks for the prompt replies :) I'll do some more digging! | 2017-11-12T14:46:03Z | [] | [] |
pypi/warehouse | 2,705 | pypi__warehouse-2705 | [
"2654",
"956"
] | 63fff23ea23ff67d3535404567c26123a93c8bb0 | diff --git a/warehouse/accounts/auth_policy.py b/warehouse/accounts/auth_policy.py
--- a/warehouse/accounts/auth_policy.py
+++ b/warehouse/accounts/auth_policy.py
@@ -34,7 +34,7 @@ def unauthenticated_userid(self, request):
# want to locate the userid from the IUserService.
if username is not None:
login_service = request.find_service(IUserService, context=None)
- return login_service.find_userid(username)
+ return str(login_service.find_userid(username))
class SessionAuthenticationPolicy(_SessionAuthenticationPolicy):
diff --git a/warehouse/accounts/views.py b/warehouse/accounts/views.py
--- a/warehouse/accounts/views.py
+++ b/warehouse/accounts/views.py
@@ -323,15 +323,6 @@ def reset_password(request, _form_class=ResetPasswordForm):
return {"form": form}
-@view_config(
- route_name="accounts.edit_gravatar",
- renderer="accounts/csi/edit_gravatar.csi.html",
- uses_session=True,
-)
-def edit_gravatar_csi(user, request):
- return {"user": user}
-
-
def _login_user(request, userid):
# We have a session factory associated with this request, so in order
# to protect against session fixation attacks we're going to make sure
diff --git a/warehouse/config.py b/warehouse/config.py
--- a/warehouse/config.py
+++ b/warehouse/config.py
@@ -362,6 +362,9 @@ def configure(settings=None):
# Register our authentication support.
config.include(".accounts")
+ # Register logged-in views
+ config.include(".manage")
+
# Allow the packaging app to register any services it has.
config.include(".packaging")
diff --git a/warehouse/manage/__init__.py b/warehouse/manage/__init__.py
new file mode 100644
--- /dev/null
+++ b/warehouse/manage/__init__.py
@@ -0,0 +1,15 @@
+# Licensed under the Apache License, Version 2.0 (the "License");
+# you may not use this file except in compliance with the License.
+# You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing, software
+# distributed under the License is distributed on an "AS IS" BASIS,
+# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+# See the License for the specific language governing permissions and
+# limitations under the License.
+
+
+def includeme(config):
+ pass
diff --git a/warehouse/manage/forms.py b/warehouse/manage/forms.py
new file mode 100644
--- /dev/null
+++ b/warehouse/manage/forms.py
@@ -0,0 +1,57 @@
+# Licensed under the Apache License, Version 2.0 (the "License");
+# you may not use this file except in compliance with the License.
+# You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing, software
+# distributed under the License is distributed on an "AS IS" BASIS,
+# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+# See the License for the specific language governing permissions and
+# limitations under the License.
+
+import wtforms
+
+from warehouse import forms
+
+
+class RoleNameMixin:
+
+ role_name = wtforms.SelectField(
+ 'Select a role',
+ choices=[
+ ('Owner', 'Owner'),
+ ('Maintainer', 'Maintainer'),
+ ],
+ validators=[
+ wtforms.validators.DataRequired(message="Must select a role"),
+ ]
+ )
+
+
+class UsernameMixin:
+
+ username = wtforms.StringField(
+ validators=[
+ wtforms.validators.DataRequired(message="Must specify a username"),
+ ]
+ )
+
+ def validate_username(self, field):
+ userid = self.user_service.find_userid(field.data)
+
+ if userid is None:
+ raise wtforms.validators.ValidationError(
+ "No user found with that username. Please try again."
+ )
+
+
+class CreateRoleForm(RoleNameMixin, UsernameMixin, forms.Form):
+
+ def __init__(self, *args, user_service, **kwargs):
+ super().__init__(*args, **kwargs)
+ self.user_service = user_service
+
+
+class ChangeRoleForm(RoleNameMixin, forms.Form):
+ pass
diff --git a/warehouse/manage/views.py b/warehouse/manage/views.py
new file mode 100644
--- /dev/null
+++ b/warehouse/manage/views.py
@@ -0,0 +1,257 @@
+# Licensed under the Apache License, Version 2.0 (the "License");
+
+# you may not use this file except in compliance with the License.
+# You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing, software
+# distributed under the License is distributed on an "AS IS" BASIS,
+# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+# See the License for the specific language governing permissions and
+# limitations under the License.
+
+from collections import defaultdict
+
+from pyramid.httpexceptions import HTTPSeeOther
+from pyramid.security import Authenticated
+from pyramid.view import view_config
+from sqlalchemy.orm.exc import NoResultFound
+
+from warehouse.accounts.interfaces import IUserService
+from warehouse.accounts.models import User
+from warehouse.manage.forms import CreateRoleForm, ChangeRoleForm
+from warehouse.packaging.models import JournalEntry, Role
+
+
+@view_config(
+ route_name="manage.profile",
+ renderer="manage/profile.html",
+ uses_session=True,
+ effective_principals=Authenticated,
+)
+def manage_profile(request):
+ return {}
+
+
+@view_config(
+ route_name="manage.projects",
+ renderer="manage/projects.html",
+ uses_session=True,
+ effective_principals=Authenticated,
+)
+def manage_projects(request):
+ return {}
+
+
+@view_config(
+ route_name="manage.project.settings",
+ renderer="manage/project.html",
+ uses_session=True,
+ permission="manage",
+ effective_principals=Authenticated,
+)
+def manage_project_settings(project, request):
+ return {"project": project}
+
+
+@view_config(
+ route_name="manage.project.roles",
+ renderer="manage/roles.html",
+ uses_session=True,
+ require_methods=False,
+ permission="manage",
+)
+def manage_project_roles(project, request, _form_class=CreateRoleForm):
+ user_service = request.find_service(IUserService, context=None)
+ form = _form_class(request.POST, user_service=user_service)
+
+ if request.method == "POST" and form.validate():
+ username = form.username.data
+ role_name = form.role_name.data
+ userid = user_service.find_userid(username)
+ user = user_service.get_user(userid)
+
+ if (request.db.query(
+ request.db.query(Role).filter(
+ Role.user == user,
+ Role.project == project,
+ Role.role_name == role_name,
+ )
+ .exists()).scalar()):
+ request.session.flash(
+ f"User '{username}' already has {role_name} role for project",
+ queue="error"
+ )
+ else:
+ request.db.add(
+ Role(user=user, project=project, role_name=form.role_name.data)
+ )
+ request.db.add(
+ JournalEntry(
+ name=project.name,
+ action=f"add {role_name} {username}",
+ submitted_by=request.user,
+ submitted_from=request.remote_addr,
+ ),
+ )
+ request.session.flash(
+ f"Added collaborator '{form.username.data}'",
+ queue="success"
+ )
+ form = _form_class(user_service=user_service)
+
+ roles = (
+ request.db.query(Role)
+ .join(User)
+ .filter(Role.project == project)
+ .all()
+ )
+
+ # TODO: The following lines are a hack to handle multiple roles for a
+ # single user and should be removed when fixing GH-2745
+ roles_by_user = defaultdict(list)
+ for role in roles:
+ roles_by_user[role.user.username].append(role)
+
+ return {
+ "project": project,
+ "roles_by_user": roles_by_user,
+ "form": form,
+ }
+
+
+@view_config(
+ route_name="manage.project.change_role",
+ uses_session=True,
+ require_methods=["POST"],
+ permission="manage",
+)
+def change_project_role(project, request, _form_class=ChangeRoleForm):
+ # TODO: This view was modified to handle deleting multiple roles for a
+ # single user and should be updated when fixing GH-2745
+
+ form = _form_class(request.POST)
+
+ if form.validate():
+ role_ids = request.POST.getall('role_id')
+
+ if len(role_ids) > 1:
+ # This user has more than one role, so just delete all the ones
+ # that aren't what we want.
+ #
+ # TODO: This branch should be removed when fixing GH-2745.
+ roles = (
+ request.db.query(Role)
+ .filter(
+ Role.id.in_(role_ids),
+ Role.project == project,
+ Role.role_name != form.role_name.data
+ )
+ .all()
+ )
+ removing_self = any(
+ role.role_name == "Owner" and role.user == request.user
+ for role in roles
+ )
+ if removing_self:
+ request.session.flash(
+ "Cannot remove yourself as Owner", queue="error"
+ )
+ else:
+ for role in roles:
+ request.db.delete(role)
+ request.db.add(
+ JournalEntry(
+ name=project.name,
+ action=f"remove {role.role_name} {role.user_name}",
+ submitted_by=request.user,
+ submitted_from=request.remote_addr,
+ ),
+ )
+ request.session.flash(
+ 'Successfully changed role', queue="success"
+ )
+ else:
+ # This user only has one role, so get it and change the type.
+ try:
+ role = (
+ request.db.query(Role)
+ .filter(
+ Role.id == request.POST.get('role_id'),
+ Role.project == project,
+ )
+ .one()
+ )
+ if role.role_name == "Owner" and role.user == request.user:
+ request.session.flash(
+ "Cannot remove yourself as Owner", queue="error"
+ )
+ else:
+ request.db.add(
+ JournalEntry(
+ name=project.name,
+ action="change {} {} to {}".format(
+ role.role_name,
+ role.user_name,
+ form.role_name.data,
+ ),
+ submitted_by=request.user,
+ submitted_from=request.remote_addr,
+ ),
+ )
+ role.role_name = form.role_name.data
+ request.session.flash(
+ 'Successfully changed role', queue="success"
+ )
+ except NoResultFound:
+ request.session.flash("Could not find role", queue="error")
+
+ return HTTPSeeOther(
+ request.route_path('manage.project.roles', name=project.name)
+ )
+
+
+@view_config(
+ route_name="manage.project.delete_role",
+ uses_session=True,
+ require_methods=["POST"],
+ permission="manage",
+)
+def delete_project_role(project, request):
+ # TODO: This view was modified to handle deleting multiple roles for a
+ # single user and should be updated when fixing GH-2745
+
+ roles = (
+ request.db.query(Role)
+ .filter(
+ Role.id.in_(request.POST.getall('role_id')),
+ Role.project == project,
+ )
+ .all()
+ )
+ removing_self = any(
+ role.role_name == "Owner" and role.user == request.user
+ for role in roles
+ )
+
+ if not roles:
+ request.session.flash("Could not find role", queue="error")
+ elif removing_self:
+ request.session.flash("Cannot remove yourself as Owner", queue="error")
+ else:
+ for role in roles:
+ request.db.delete(role)
+ request.db.add(
+ JournalEntry(
+ name=project.name,
+ action=f"remove {role.role_name} {role.user_name}",
+ submitted_by=request.user,
+ submitted_from=request.remote_addr,
+ ),
+ )
+ request.session.flash("Successfully removed role", queue="success")
+
+ return HTTPSeeOther(
+ request.route_path('manage.project.roles', name=project.name)
+ )
diff --git a/warehouse/packaging/models.py b/warehouse/packaging/models.py
--- a/warehouse/packaging/models.py
+++ b/warehouse/packaging/models.py
@@ -59,6 +59,16 @@ class Role(db.Model):
user = orm.relationship(User, lazy=False)
project = orm.relationship("Project", lazy=False)
+ def __gt__(self, other):
+ '''
+ Temporary hack to allow us to only display the 'highest' role when
+ there are multiple for a given user
+
+ TODO: This should be removed when fixing GH-2745.
+ '''
+ order = ['Maintainer', 'Owner'] # from lowest to highest
+ return order.index(self.role_name) > order.index(other.role_name)
+
class ProjectFactory:
@@ -147,8 +157,10 @@ def __acl__(self):
for role in sorted(
query.all(),
key=lambda x: ["Owner", "Maintainer"].index(x.role_name)):
- acls.append((Allow, role.user.id, ["upload"]))
-
+ if role.role_name == "Owner":
+ acls.append((Allow, str(role.user.id), ["manage", "upload"]))
+ else:
+ acls.append((Allow, str(role.user.id), ["upload"]))
return acls
@property
diff --git a/warehouse/routes.py b/warehouse/routes.py
--- a/warehouse/routes.py
+++ b/warehouse/routes.py
@@ -97,11 +97,36 @@ def includeme(config):
"/account/reset-password/",
domain=warehouse,
)
+
+ # Management (views for logged-in users)
+ config.add_route("manage.profile", "/manage/profile/", domain=warehouse)
+ config.add_route("manage.projects", "/manage/projects/", domain=warehouse)
config.add_route(
- "accounts.edit_gravatar",
- "/user/{username}/edit_gravatar/",
- factory="warehouse.accounts.models:UserFactory",
- traverse="/{username}",
+ "manage.project.settings",
+ "/manage/project/{name}/settings/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{name}",
+ domain=warehouse,
+ )
+ config.add_route(
+ "manage.project.roles",
+ "/manage/project/{name}/collaboration/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{name}",
+ domain=warehouse,
+ )
+ config.add_route(
+ "manage.project.change_role",
+ "/manage/project/{name}/collaboration/change/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{name}",
+ domain=warehouse,
+ )
+ config.add_route(
+ "manage.project.delete_role",
+ "/manage/project/{name}/collaboration/delete/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{name}",
domain=warehouse,
)
diff --git a/warehouse/views.py b/warehouse/views.py
--- a/warehouse/views.py
+++ b/warehouse/views.py
@@ -16,10 +16,12 @@
HTTPException, HTTPSeeOther, HTTPMovedPermanently, HTTPNotFound,
HTTPBadRequest, exception_response,
)
+from pyramid.exceptions import PredicateMismatch
from pyramid.renderers import render_to_response
from pyramid.response import Response
from pyramid.view import (
- notfound_view_config, forbidden_view_config, view_config,
+ notfound_view_config, forbidden_view_config, exception_view_config,
+ view_config,
)
from elasticsearch_dsl import Q
from sqlalchemy import func
@@ -107,6 +109,7 @@ def httpexception_view(exc, request):
@forbidden_view_config()
+@exception_view_config(PredicateMismatch)
def forbidden(exc, request, redirect_to="accounts.login"):
# If the forbidden error is because the user isn't logged in, then we'll
# redirect them to the log in page.
| diff --git a/tests/unit/accounts/test_auth_policy.py b/tests/unit/accounts/test_auth_policy.py
--- a/tests/unit/accounts/test_auth_policy.py
+++ b/tests/unit/accounts/test_auth_policy.py
@@ -11,6 +11,7 @@
# limitations under the License.
import pretend
+import uuid
from pyramid import authentication
from pyramid.interfaces import IAuthenticationPolicy
@@ -74,7 +75,7 @@ def test_unauthenticated_userid_with_userid(self, monkeypatch):
add_vary_cb = pretend.call_recorder(lambda *v: vary_cb)
monkeypatch.setattr(auth_policy, "add_vary_callback", add_vary_cb)
- userid = pretend.stub()
+ userid = uuid.uuid4()
service = pretend.stub(
find_userid=pretend.call_recorder(lambda username: userid),
)
@@ -83,7 +84,7 @@ def test_unauthenticated_userid_with_userid(self, monkeypatch):
add_response_callback=pretend.call_recorder(lambda cb: None),
)
- assert policy.unauthenticated_userid(request) is userid
+ assert policy.unauthenticated_userid(request) == str(userid)
assert extract_http_basic_credentials.calls == [pretend.call(request)]
assert request.find_service.calls == [
pretend.call(IUserService, context=None),
diff --git a/tests/unit/accounts/test_views.py b/tests/unit/accounts/test_views.py
--- a/tests/unit/accounts/test_views.py
+++ b/tests/unit/accounts/test_views.py
@@ -559,15 +559,6 @@ def test_reset_password(self, db_request, user_service, token_service):
]
-class TestClientSideIncludes:
-
- def test_edit_gravatar_csi_returns_user(self, db_request):
- user = UserFactory.create()
- assert views.edit_gravatar_csi(user, db_request) == {
- "user": user,
- }
-
-
class TestProfileCallout:
def test_profile_callout_returns_user(self):
diff --git a/tests/unit/manage/__init__.py b/tests/unit/manage/__init__.py
new file mode 100644
--- /dev/null
+++ b/tests/unit/manage/__init__.py
@@ -0,0 +1,11 @@
+# Licensed under the Apache License, Version 2.0 (the "License");
+# you may not use this file except in compliance with the License.
+# You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing, software
+# distributed under the License is distributed on an "AS IS" BASIS,
+# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+# See the License for the specific language governing permissions and
+# limitations under the License.
diff --git a/tests/unit/manage/test_forms.py b/tests/unit/manage/test_forms.py
new file mode 100644
--- /dev/null
+++ b/tests/unit/manage/test_forms.py
@@ -0,0 +1,71 @@
+# Licensed under the Apache License, Version 2.0 (the "License");
+# you may not use this file except in compliance with the License.
+# You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing, software
+# distributed under the License is distributed on an "AS IS" BASIS,
+# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+# See the License for the specific language governing permissions and
+# limitations under the License.
+
+import pretend
+import pytest
+import wtforms
+
+from webob.multidict import MultiDict
+
+from warehouse.manage import forms
+
+
+class TestCreateRoleForm:
+
+ def test_creation(self):
+ user_service = pretend.stub()
+ form = forms.CreateRoleForm(user_service=user_service)
+
+ assert form.user_service is user_service
+
+ def test_validate_username_with_no_user(self):
+ user_service = pretend.stub(
+ find_userid=pretend.call_recorder(lambda userid: None),
+ )
+ form = forms.CreateRoleForm(user_service=user_service)
+ field = pretend.stub(data="my_username")
+
+ with pytest.raises(wtforms.validators.ValidationError):
+ form.validate_username(field)
+
+ assert user_service.find_userid.calls == [pretend.call("my_username")]
+
+ def test_validate_username_with_user(self):
+ user_service = pretend.stub(
+ find_userid=pretend.call_recorder(lambda userid: 1),
+ )
+ form = forms.CreateRoleForm(user_service=user_service)
+ field = pretend.stub(data="my_username")
+
+ form.validate_username(field)
+
+ assert user_service.find_userid.calls == [pretend.call("my_username")]
+
+ @pytest.mark.parametrize(("value", "expected"), [
+ ("", "Must select a role"),
+ ("invalid", "Not a valid choice"),
+ (None, "Not a valid choice"),
+ ])
+ def test_validate_role_name_fails(self, value, expected):
+ user_service = pretend.stub(
+ find_userid=pretend.call_recorder(lambda userid: 1),
+ )
+ form = forms.CreateRoleForm(
+ MultiDict({
+ 'role_name': value,
+ 'username': 'valid_username',
+ }),
+ user_service=user_service,
+ )
+
+ assert not form.validate()
+ assert form.role_name.errors == [expected]
diff --git a/tests/unit/manage/test_views.py b/tests/unit/manage/test_views.py
new file mode 100644
--- /dev/null
+++ b/tests/unit/manage/test_views.py
@@ -0,0 +1,505 @@
+# Licensed under the Apache License, Version 2.0 (the "License");
+# you may not use this file except in compliance with the License.
+# You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing, software
+# distributed under the License is distributed on an "AS IS" BASIS,
+# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+# See the License for the specific language governing permissions and
+# limitations under the License.
+
+import uuid
+
+import pretend
+
+from pyramid.httpexceptions import HTTPSeeOther
+from webob.multidict import MultiDict
+
+from warehouse.manage import views
+from warehouse.accounts.interfaces import IUserService
+from warehouse.packaging.models import JournalEntry, Role
+
+from ...common.db.packaging import ProjectFactory, RoleFactory, UserFactory
+
+
+class TestManageProfile:
+
+ def test_manage_profile(self):
+ request = pretend.stub()
+
+ assert views.manage_profile(request) == {}
+
+
+class TestManageProjects:
+
+ def test_manage_projects(self):
+ request = pretend.stub()
+
+ assert views.manage_projects(request) == {}
+
+
+class TestManageProjectSettings:
+
+ def test_manage_project_settings(self):
+ request = pretend.stub()
+ project = pretend.stub()
+
+ assert views.manage_project_settings(project, request) == {
+ "project": project,
+ }
+
+
+class TestManageProjectRoles:
+
+ def test_get_manage_project_roles(self, db_request):
+ user_service = pretend.stub()
+ db_request.find_service = pretend.call_recorder(
+ lambda iface, context: user_service
+ )
+ form_obj = pretend.stub()
+ form_class = pretend.call_recorder(lambda d, user_service: form_obj)
+
+ project = ProjectFactory.create(name="foobar")
+ user = UserFactory.create()
+ role = RoleFactory.create(user=user, project=project)
+
+ result = views.manage_project_roles(
+ project, db_request, _form_class=form_class
+ )
+
+ assert db_request.find_service.calls == [
+ pretend.call(IUserService, context=None),
+ ]
+ assert form_class.calls == [
+ pretend.call(db_request.POST, user_service=user_service),
+ ]
+ assert result == {
+
+ "project": project,
+ "roles_by_user": {user.username: [role]},
+ "form": form_obj,
+ }
+
+ def test_post_new_role_validation_fails(self, db_request):
+ project = ProjectFactory.create(name="foobar")
+ user = UserFactory.create(username="testuser")
+ role = RoleFactory.create(user=user, project=project)
+
+ user_service = pretend.stub()
+ db_request.find_service = pretend.call_recorder(
+ lambda iface, context: user_service
+ )
+ db_request.method = "POST"
+ form_obj = pretend.stub(validate=pretend.call_recorder(lambda: False))
+ form_class = pretend.call_recorder(lambda d, user_service: form_obj)
+
+ result = views.manage_project_roles(
+ project, db_request, _form_class=form_class
+ )
+
+ assert db_request.find_service.calls == [
+ pretend.call(IUserService, context=None),
+ ]
+ assert form_class.calls == [
+ pretend.call(db_request.POST, user_service=user_service),
+ ]
+ assert form_obj.validate.calls == [pretend.call()]
+ assert result == {
+ "project": project,
+ "roles_by_user": {user.username: [role]},
+ "form": form_obj,
+ }
+
+ def test_post_new_role(self, db_request):
+ project = ProjectFactory.create(name="foobar")
+ user = UserFactory.create(username="testuser")
+
+ user_service = pretend.stub(
+ find_userid=lambda username: user.id,
+ get_user=lambda userid: user,
+ )
+ db_request.find_service = pretend.call_recorder(
+ lambda iface, context: user_service
+ )
+ db_request.method = "POST"
+ db_request.POST = pretend.stub()
+ db_request.remote_addr = "10.10.10.10"
+ db_request.user = UserFactory.create()
+ form_obj = pretend.stub(
+ validate=pretend.call_recorder(lambda: True),
+ username=pretend.stub(data=user.username),
+ role_name=pretend.stub(data="Owner"),
+ )
+ form_class = pretend.call_recorder(lambda *a, **kw: form_obj)
+ db_request.session = pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ )
+
+ result = views.manage_project_roles(
+ project, db_request, _form_class=form_class
+ )
+
+ assert db_request.find_service.calls == [
+ pretend.call(IUserService, context=None),
+ ]
+ assert form_obj.validate.calls == [pretend.call()]
+ assert form_class.calls == [
+ pretend.call(db_request.POST, user_service=user_service),
+ pretend.call(user_service=user_service),
+ ]
+ assert db_request.session.flash.calls == [
+ pretend.call("Added collaborator 'testuser'", queue="success"),
+ ]
+
+ # Only one role is created
+ role = db_request.db.query(Role).one()
+
+ assert result == {
+ "project": project,
+ "roles_by_user": {user.username: [role]},
+ "form": form_obj,
+ }
+
+ entry = db_request.db.query(JournalEntry).one()
+
+ assert entry.name == project.name
+ assert entry.action == "add Owner testuser"
+ assert entry.submitted_by == db_request.user
+ assert entry.submitted_from == db_request.remote_addr
+
+ def test_post_duplicate_role(self, db_request):
+ project = ProjectFactory.create(name="foobar")
+ user = UserFactory.create(username="testuser")
+ role = RoleFactory.create(
+ user=user, project=project, role_name="Owner"
+ )
+
+ user_service = pretend.stub(
+ find_userid=lambda username: user.id,
+ get_user=lambda userid: user,
+ )
+ db_request.find_service = pretend.call_recorder(
+ lambda iface, context: user_service
+ )
+ db_request.method = "POST"
+ db_request.POST = pretend.stub()
+ form_obj = pretend.stub(
+ validate=pretend.call_recorder(lambda: True),
+ username=pretend.stub(data=user.username),
+ role_name=pretend.stub(data=role.role_name),
+ )
+ form_class = pretend.call_recorder(lambda *a, **kw: form_obj)
+ db_request.session = pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ )
+
+ result = views.manage_project_roles(
+ project, db_request, _form_class=form_class
+ )
+
+ assert db_request.find_service.calls == [
+ pretend.call(IUserService, context=None),
+ ]
+ assert form_obj.validate.calls == [pretend.call()]
+ assert form_class.calls == [
+ pretend.call(db_request.POST, user_service=user_service),
+ pretend.call(user_service=user_service),
+ ]
+ assert db_request.session.flash.calls == [
+ pretend.call(
+ "User 'testuser' already has Owner role for project",
+ queue="error",
+ ),
+ ]
+
+ # No additional roles are created
+ assert role == db_request.db.query(Role).one()
+
+ assert result == {
+ "project": project,
+ "roles_by_user": {user.username: [role]},
+ "form": form_obj,
+ }
+
+
+class TestChangeProjectRoles:
+
+ def test_change_role(self, db_request):
+ project = ProjectFactory.create(name="foobar")
+ user = UserFactory.create(username="testuser")
+ role = RoleFactory.create(
+ user=user, project=project, role_name="Owner"
+ )
+ new_role_name = "Maintainer"
+
+ db_request.method = "POST"
+ db_request.user = UserFactory.create()
+ db_request.remote_addr = "10.10.10.10"
+ db_request.POST = MultiDict({
+ "role_id": role.id,
+ "role_name": new_role_name,
+ })
+ db_request.session = pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ )
+ db_request.route_path = pretend.call_recorder(
+ lambda *a, **kw: "/the-redirect"
+ )
+
+ result = views.change_project_role(project, db_request)
+
+ assert role.role_name == new_role_name
+ assert db_request.route_path.calls == [
+ pretend.call('manage.project.roles', name=project.name),
+ ]
+ assert db_request.session.flash.calls == [
+ pretend.call("Successfully changed role", queue="success"),
+ ]
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/the-redirect"
+
+ entry = db_request.db.query(JournalEntry).one()
+
+ assert entry.name == project.name
+ assert entry.action == "change Owner testuser to Maintainer"
+ assert entry.submitted_by == db_request.user
+ assert entry.submitted_from == db_request.remote_addr
+
+ def test_change_role_invalid_role_name(self, pyramid_request):
+ project = pretend.stub(name="foobar")
+
+ pyramid_request.method = "POST"
+ pyramid_request.POST = MultiDict({
+ "role_id": str(uuid.uuid4()),
+ "role_name": "Invalid Role Name",
+ })
+ pyramid_request.route_path = pretend.call_recorder(
+ lambda *a, **kw: "/the-redirect"
+ )
+
+ result = views.change_project_role(project, pyramid_request)
+
+ assert pyramid_request.route_path.calls == [
+ pretend.call('manage.project.roles', name=project.name),
+ ]
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/the-redirect"
+
+ def test_change_role_when_multiple(self, db_request):
+ project = ProjectFactory.create(name="foobar")
+ user = UserFactory.create(username="testuser")
+ owner_role = RoleFactory.create(
+ user=user, project=project, role_name="Owner"
+ )
+ maintainer_role = RoleFactory.create(
+ user=user, project=project, role_name="Maintainer"
+ )
+ new_role_name = "Maintainer"
+
+ db_request.method = "POST"
+ db_request.user = UserFactory.create()
+ db_request.remote_addr = "10.10.10.10"
+ db_request.POST = MultiDict([
+ ("role_id", owner_role.id),
+ ("role_id", maintainer_role.id),
+ ("role_name", new_role_name),
+ ])
+ db_request.session = pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ )
+ db_request.route_path = pretend.call_recorder(
+ lambda *a, **kw: "/the-redirect"
+ )
+
+ result = views.change_project_role(project, db_request)
+
+ assert db_request.db.query(Role).all() == [maintainer_role]
+ assert db_request.route_path.calls == [
+ pretend.call('manage.project.roles', name=project.name),
+ ]
+ assert db_request.session.flash.calls == [
+ pretend.call("Successfully changed role", queue="success"),
+ ]
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/the-redirect"
+
+ entry = db_request.db.query(JournalEntry).one()
+
+ assert entry.name == project.name
+ assert entry.action == "remove Owner testuser"
+ assert entry.submitted_by == db_request.user
+ assert entry.submitted_from == db_request.remote_addr
+
+ def test_change_missing_role(self, db_request):
+ project = ProjectFactory.create(name="foobar")
+ missing_role_id = str(uuid.uuid4())
+
+ db_request.method = "POST"
+ db_request.user = pretend.stub()
+ db_request.POST = MultiDict({
+ "role_id": missing_role_id,
+ "role_name": 'Owner',
+ })
+ db_request.session = pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ )
+ db_request.route_path = pretend.call_recorder(
+ lambda *a, **kw: "/the-redirect"
+ )
+
+ result = views.change_project_role(project, db_request)
+
+ assert db_request.session.flash.calls == [
+ pretend.call("Could not find role", queue="error"),
+ ]
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/the-redirect"
+
+ def test_change_own_owner_role(self, db_request):
+ project = ProjectFactory.create(name="foobar")
+ user = UserFactory.create(username="testuser")
+ role = RoleFactory.create(
+ user=user, project=project, role_name="Owner"
+ )
+
+ db_request.method = "POST"
+ db_request.user = user
+ db_request.POST = MultiDict({
+ "role_id": role.id,
+ "role_name": "Maintainer",
+ })
+ db_request.session = pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ )
+ db_request.route_path = pretend.call_recorder(
+ lambda *a, **kw: "/the-redirect"
+ )
+
+ result = views.change_project_role(project, db_request)
+
+ assert db_request.session.flash.calls == [
+ pretend.call("Cannot remove yourself as Owner", queue="error"),
+ ]
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/the-redirect"
+
+ def test_change_own_owner_role_when_multiple(self, db_request):
+ project = ProjectFactory.create(name="foobar")
+ user = UserFactory.create(username="testuser")
+ owner_role = RoleFactory.create(
+ user=user, project=project, role_name="Owner"
+ )
+ maintainer_role = RoleFactory.create(
+ user=user, project=project, role_name="Maintainer"
+ )
+
+ db_request.method = "POST"
+ db_request.user = user
+ db_request.POST = MultiDict([
+ ("role_id", owner_role.id),
+ ("role_id", maintainer_role.id),
+ ("role_name", "Maintainer"),
+ ])
+ db_request.session = pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ )
+ db_request.route_path = pretend.call_recorder(
+ lambda *a, **kw: "/the-redirect"
+ )
+
+ result = views.change_project_role(project, db_request)
+
+ assert db_request.session.flash.calls == [
+ pretend.call("Cannot remove yourself as Owner", queue="error"),
+ ]
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/the-redirect"
+
+
+class TestDeleteProjectRoles:
+
+ def test_delete_role(self, db_request):
+ project = ProjectFactory.create(name="foobar")
+ user = UserFactory.create(username="testuser")
+ role = RoleFactory.create(
+ user=user, project=project, role_name="Owner"
+ )
+
+ db_request.method = "POST"
+ db_request.user = UserFactory.create()
+ db_request.remote_addr = "10.10.10.10"
+ db_request.POST = MultiDict({"role_id": role.id})
+ db_request.session = pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ )
+ db_request.route_path = pretend.call_recorder(
+ lambda *a, **kw: "/the-redirect"
+ )
+
+ result = views.delete_project_role(project, db_request)
+
+ assert db_request.route_path.calls == [
+ pretend.call('manage.project.roles', name=project.name),
+ ]
+ assert db_request.db.query(Role).all() == []
+ assert db_request.session.flash.calls == [
+ pretend.call("Successfully removed role", queue="success"),
+ ]
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/the-redirect"
+
+ entry = db_request.db.query(JournalEntry).one()
+
+ assert entry.name == project.name
+ assert entry.action == "remove Owner testuser"
+ assert entry.submitted_by == db_request.user
+ assert entry.submitted_from == db_request.remote_addr
+
+ def test_delete_missing_role(self, db_request):
+ project = ProjectFactory.create(name="foobar")
+ missing_role_id = str(uuid.uuid4())
+
+ db_request.method = "POST"
+ db_request.user = pretend.stub()
+ db_request.POST = MultiDict({"role_id": missing_role_id})
+ db_request.session = pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ )
+ db_request.route_path = pretend.call_recorder(
+ lambda *a, **kw: "/the-redirect"
+ )
+
+ result = views.delete_project_role(project, db_request)
+
+ assert db_request.session.flash.calls == [
+ pretend.call("Could not find role", queue="error"),
+ ]
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/the-redirect"
+
+ def test_delete_own_owner_role(self, db_request):
+ project = ProjectFactory.create(name="foobar")
+ user = UserFactory.create(username="testuser")
+ role = RoleFactory.create(
+ user=user, project=project, role_name="Owner"
+ )
+
+ db_request.method = "POST"
+ db_request.user = user
+ db_request.POST = MultiDict({"role_id": role.id})
+ db_request.session = pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ )
+ db_request.route_path = pretend.call_recorder(
+ lambda *a, **kw: "/the-redirect"
+ )
+
+ result = views.delete_project_role(project, db_request)
+
+ assert db_request.session.flash.calls == [
+ pretend.call("Cannot remove yourself as Owner", queue="error"),
+ ]
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/the-redirect"
diff --git a/tests/unit/packaging/test_models.py b/tests/unit/packaging/test_models.py
--- a/tests/unit/packaging/test_models.py
+++ b/tests/unit/packaging/test_models.py
@@ -27,6 +27,21 @@
)
+class TestRole:
+
+ def test_role_ordering(self, db_request):
+ project = DBProjectFactory.create()
+ owner_role = DBRoleFactory.create(
+ project=project,
+ role_name="Owner",
+ )
+ maintainer_role = DBRoleFactory.create(
+ project=project,
+ role_name="Maintainer",
+ )
+ assert max([maintainer_role, owner_role]) == owner_role
+
+
class TestProjectFactory:
@pytest.mark.parametrize(
@@ -95,10 +110,10 @@ def test_acl(self, db_session):
assert project.__acl__() == [
(Allow, "group:admins", "admin"),
- (Allow, owner1.user.id, ["upload"]),
- (Allow, owner2.user.id, ["upload"]),
- (Allow, maintainer1.user.id, ["upload"]),
- (Allow, maintainer2.user.id, ["upload"]),
+ (Allow, str(owner1.user.id), ["manage", "upload"]),
+ (Allow, str(owner2.user.id), ["manage", "upload"]),
+ (Allow, str(maintainer1.user.id), ["upload"]),
+ (Allow, str(maintainer2.user.id), ["upload"]),
]
diff --git a/tests/unit/test_config.py b/tests/unit/test_config.py
--- a/tests/unit/test_config.py
+++ b/tests/unit/test_config.py
@@ -336,6 +336,7 @@ def __init__(self):
pretend.call(".cache.http"),
pretend.call(".cache.origin"),
pretend.call(".accounts"),
+ pretend.call(".manage"),
pretend.call(".packaging"),
pretend.call(".redirects"),
pretend.call(".routes"),
diff --git a/tests/unit/test_routes.py b/tests/unit/test_routes.py
--- a/tests/unit/test_routes.py
+++ b/tests/unit/test_routes.py
@@ -126,10 +126,41 @@ def add_policy(name, filename):
domain=warehouse,
),
pretend.call(
- "accounts.edit_gravatar",
- "/user/{username}/edit_gravatar/",
- factory="warehouse.accounts.models:UserFactory",
- traverse="/{username}",
+ "manage.profile",
+ "/manage/profile/",
+ domain=warehouse
+ ),
+ pretend.call(
+ "manage.projects",
+ "/manage/projects/",
+ domain=warehouse
+ ),
+ pretend.call(
+ "manage.project.settings",
+ "/manage/project/{name}/settings/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{name}",
+ domain=warehouse,
+ ),
+ pretend.call(
+ "manage.project.roles",
+ "/manage/project/{name}/collaboration/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{name}",
+ domain=warehouse,
+ ),
+ pretend.call(
+ "manage.project.change_role",
+ "/manage/project/{name}/collaboration/change/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{name}",
+ domain=warehouse,
+ ),
+ pretend.call(
+ "manage.project.delete_role",
+ "/manage/project/{name}/collaboration/delete/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{name}",
domain=warehouse,
),
pretend.call(
| Role maintenance for package owners
Owners need to be able to add "Maintainer"/"Owner" roles to the packages they own.
Legacy:
<img width="878" alt="screen shot 2017-12-08 at 11 45 57 am" src="https://user-images.githubusercontent.com/294415/33778215-6745c8bc-dc0d-11e7-9c14-5ee69c49547c.png">
[Feature Request] UI for adding maintainers
The idea here is simple, but I have no clue how hard it would be to implement. That said, it would be great if warehouse had a user interface for current maintainers of a project to add other maintainers.
| I had this issue today, actually: spent several minutes hunting for the option before I realized I had to go back to the old website to update roles.
Until this is fixed, could we get something added to the "Common Questions" redirecting people to pypi.python.org ?
Hi @ehthiede, sorry that happened to you!
We have https://github.com/pypa/warehouse/issues/2659 already which should capture this. Additionally, we're planning to ship this milestone in the upcoming weeks, so this specific issue shouldn't be missing for much longer.
@di Not a problem! Just felt stupid for a bit. Thanks for the prompt update!
-Erik
@dstufft Need help?
Contributions are always welcome :)
As this is a new feature, I'm going to move this out of the 'shutdown' milestone and label it with post launch high priority.
This isn't a new feature, it exists on PyPI today, people can add/remove Owners and Maintainers and it's needed before we shut down legacy or else people won't be able to manage the permissions of their projects.
| 2017-12-26T20:11:49Z | [] | [] |
pypi/warehouse | 2,764 | pypi__warehouse-2764 | [
"1228"
] | 45da118b6918b5f93334b10fb22dbf07ba497dbd | diff --git a/warehouse/accounts/__init__.py b/warehouse/accounts/__init__.py
--- a/warehouse/accounts/__init__.py
+++ b/warehouse/accounts/__init__.py
@@ -15,8 +15,10 @@
from pyramid.authorization import ACLAuthorizationPolicy
from pyramid_multiauth import MultiAuthenticationPolicy
-from warehouse.accounts.interfaces import IUserService
-from warehouse.accounts.services import database_login_factory
+from warehouse.accounts.interfaces import IUserService, IUserTokenService
+from warehouse.accounts.services import (
+ database_login_factory, user_token_factory
+)
from warehouse.accounts.auth_policy import (
BasicAuthAuthenticationPolicy, SessionAuthenticationPolicy,
)
@@ -66,6 +68,7 @@ def _user(request):
def includeme(config):
# Register our login service
config.register_service_factory(database_login_factory, IUserService)
+ config.register_service_factory(user_token_factory, IUserTokenService)
# Register our authentication and authorization policies
config.set_authentication_policy(
diff --git a/warehouse/accounts/forms.py b/warehouse/accounts/forms.py
--- a/warehouse/accounts/forms.py
+++ b/warehouse/accounts/forms.py
@@ -18,7 +18,25 @@
from warehouse.accounts.interfaces import TooManyFailedLogins
-class CredentialsMixin:
+class UsernameMixin:
+
+ username = wtforms.StringField(
+ validators=[
+ wtforms.validators.DataRequired(),
+ ],
+ )
+
+ def validate_username(self, field):
+ userid = self.user_service.find_userid(field.data)
+
+ if userid is None:
+ raise wtforms.validators.ValidationError(
+ "No user found with that username."
+ )
+
+
+class NewUsernameMixin:
+
username = wtforms.StringField(
validators=[
wtforms.validators.DataRequired(),
@@ -44,18 +62,25 @@ class CredentialsMixin:
],
)
+ def validate_username(self, field):
+ if self.user_service.find_userid(field.data) is not None:
+ raise wtforms.validators.ValidationError(
+ "This username is already being used by another "
+ "account. Please choose a different username."
+ )
+
+
+class PasswordMixin:
+
password = wtforms.PasswordField(
validators=[
wtforms.validators.DataRequired(),
],
)
- def __init__(self, *args, user_service, **kwargs):
- super().__init__(*args, **kwargs)
- self.user_service = user_service
+class NewPasswordMixin:
-class RegistrationForm(CredentialsMixin, forms.Form):
password = wtforms.PasswordField(
validators=[
wtforms.validators.DataRequired(),
@@ -64,6 +89,7 @@ class RegistrationForm(CredentialsMixin, forms.Form):
),
],
)
+
password_confirm = wtforms.PasswordField(
validators=[
wtforms.validators.DataRequired(),
@@ -73,6 +99,9 @@ class RegistrationForm(CredentialsMixin, forms.Form):
],
)
+
+class RegistrationForm(NewPasswordMixin, NewUsernameMixin, forms.Form):
+
full_name = wtforms.StringField()
email = wtforms.fields.html5.EmailField(
@@ -89,17 +118,11 @@ class RegistrationForm(CredentialsMixin, forms.Form):
g_recaptcha_response = wtforms.StringField()
- def __init__(self, *args, recaptcha_service, **kwargs):
+ def __init__(self, *args, recaptcha_service, user_service, **kwargs):
super().__init__(*args, **kwargs)
+ self.user_service = user_service
self.recaptcha_service = recaptcha_service
- def validate_username(self, field):
- if self.user_service.find_userid(field.data) is not None:
- raise wtforms.validators.ValidationError(
- "This username is already being used by another "
- "account. Please choose a different username."
- )
-
def validate_email(self, field):
if self.user_service.find_userid_by_email(field.data) is not None:
raise wtforms.validators.ValidationError(
@@ -125,14 +148,11 @@ def validate_g_recaptcha_response(self, field):
raise wtforms.validators.ValidationError("Recaptcha error.")
-class LoginForm(CredentialsMixin, forms.Form):
- def validate_username(self, field):
- userid = self.user_service.find_userid(field.data)
+class LoginForm(PasswordMixin, UsernameMixin, forms.Form):
- if userid is None:
- raise wtforms.validators.ValidationError(
- "No user found with that username. Please try again."
- )
+ def __init__(self, *args, user_service, **kwargs):
+ super().__init__(*args, **kwargs)
+ self.user_service = user_service
def validate_password(self, field):
userid = self.user_service.find_userid(self.username.data)
@@ -148,3 +168,20 @@ def validate_password(self, field):
"There have been too many unsuccessful login attempts, "
"please try again later."
) from None
+
+
+class RequestPasswordResetForm(UsernameMixin, forms.Form):
+
+ def __init__(self, *args, user_service, **kwargs):
+ super().__init__(*args, **kwargs)
+ self.user_service = user_service
+
+
+class ResetPasswordForm(NewPasswordMixin, forms.Form):
+
+ # These fields are here to provide the various user-defined fields to the
+ # PasswordStrengthValidator of the NewPasswordMixin, to ensure that the
+ # newly set password doesn't contain any of them
+ full_name = wtforms.StringField()
+ username = wtforms.StringField()
+ email = wtforms.StringField()
diff --git a/warehouse/accounts/interfaces.py b/warehouse/accounts/interfaces.py
--- a/warehouse/accounts/interfaces.py
+++ b/warehouse/accounts/interfaces.py
@@ -21,6 +21,13 @@ def __init__(self, *args, resets_in, **kwargs):
return super().__init__(*args, **kwargs)
+class InvalidPasswordResetToken(Exception):
+ def __init__(self, message, *args, **kwargs):
+ self.message = message
+
+ return super().__init__(*args, **kwargs)
+
+
class IUserService(Interface):
def get_user(userid):
@@ -29,6 +36,12 @@ def get_user(userid):
there is no user for that ID.
"""
+ def get_user_by_username(username):
+ """
+ Return the user object corresponding with the given username, or None
+ if there is no user with that username.
+ """
+
def find_userid(username):
"""
Find the unique user identifier for the given username or None if there
@@ -59,3 +72,16 @@ def verify_email(user_id, email_address):
"""
verifies the user
"""
+
+
+class IUserTokenService(Interface):
+
+ def generate_token(user):
+ """
+ Generates a unique token based on user attributes
+ """
+
+ def get_user_by_token(token):
+ """
+ Gets the user corresponding to the token provided
+ """
diff --git a/warehouse/accounts/services.py b/warehouse/accounts/services.py
--- a/warehouse/accounts/services.py
+++ b/warehouse/accounts/services.py
@@ -11,17 +11,24 @@
# limitations under the License.
import collections
-import datetime
import functools
+import hmac
import logging
+import uuid
from passlib.context import CryptContext
from sqlalchemy.orm.exc import NoResultFound
from zope.interface import implementer
-from warehouse.accounts.interfaces import IUserService, TooManyFailedLogins
+from warehouse.accounts.interfaces import (
+ InvalidPasswordResetToken, IUserService, IUserTokenService,
+ TooManyFailedLogins,
+)
from warehouse.accounts.models import Email, User
from warehouse.rate_limiting import IRateLimiter, DummyRateLimiter
+from warehouse.utils.crypto import (
+ BadData, SignatureExpired, URLSafeTimedSerializer,
+)
logger = logging.getLogger(__name__)
@@ -56,6 +63,7 @@ def __init__(self, session, ratelimiters=None):
argon2__time_cost=6,
)
+
@functools.lru_cache()
def get_user(self, userid):
# TODO: We probably don't actually want to just return the database
@@ -63,6 +71,11 @@ def get_user(self, userid):
# TODO: We need some sort of Anonymous User.
return self.db.query(User).get(userid)
+ @functools.lru_cache()
+ def get_user_by_username(self, username):
+ user_id = self.find_userid(username)
+ return None if user_id is None else self.get_user(user_id)
+
@functools.lru_cache()
def find_userid(self, username):
try:
@@ -167,6 +180,63 @@ def verify_email(self, user_id, email_address):
email.verified = True
+@implementer(IUserTokenService)
+class UserTokenService:
+ def __init__(self, user_service, settings):
+ self.user_service = user_service
+ self.serializer = URLSafeTimedSerializer(
+ settings["password_reset.secret"],
+ salt="password-reset",
+ )
+ self.token_max_age = settings["password_reset.token_max_age"]
+
+ def generate_token(self, user):
+ return self.serializer.dumps({
+ "user.id": str(user.id),
+ "user.last_login": str(user.last_login),
+ "user.password_date": str(user.password_date),
+ })
+
+ def get_user_by_token(self, token):
+ if not token:
+ raise InvalidPasswordResetToken(
+ "Invalid token - No token supplied"
+ )
+
+ try:
+ data = self.serializer.loads(token, max_age=self.token_max_age)
+ except SignatureExpired:
+ raise InvalidPasswordResetToken(
+ "Expired token - Token is expired, request a new password "
+ "reset link"
+ )
+ except BadData: # Catch all other exceptions
+ raise InvalidPasswordResetToken(
+ "Invalid token - Request a new password reset link"
+ )
+
+ # Check whether a user with the given user ID exists
+ user = self.user_service.get_user(uuid.UUID(data.get("user.id")))
+ if user is None:
+ raise InvalidPasswordResetToken("Invalid token - User not found")
+
+ last_login = data.get("user.last_login")
+ if str(user.last_login) > last_login:
+ raise InvalidPasswordResetToken(
+ "Invalid token - User has logged in since this token was "
+ "requested"
+ ) # TODO: track and audit this, seems alertable
+
+ password_date = data.get("user.password_date")
+ if str(user.password_date) > password_date:
+ raise InvalidPasswordResetToken(
+ "Invalid token - Password has already been changed since this "
+ "token was requested"
+ )
+
+ return user
+
+
def database_login_factory(context, request):
return DatabaseUserService(
request.db,
@@ -183,3 +253,10 @@ def database_login_factory(context, request):
),
},
)
+
+
+def user_token_factory(context, request):
+ return UserTokenService(
+ request.find_service(IUserService),
+ request.registry.settings
+ )
diff --git a/warehouse/accounts/views.py b/warehouse/accounts/views.py
--- a/warehouse/accounts/views.py
+++ b/warehouse/accounts/views.py
@@ -16,14 +16,21 @@
from pyramid.httpexceptions import (
HTTPMovedPermanently, HTTPSeeOther, HTTPTooManyRequests,
)
+from pyramid.renderers import render
from pyramid.security import remember, forget
from pyramid.view import view_config
from sqlalchemy.orm import joinedload
from warehouse.accounts import REDIRECT_FIELD_NAME
-from warehouse.accounts import forms
-from warehouse.accounts.interfaces import IUserService, TooManyFailedLogins
+from warehouse.accounts.forms import (
+ LoginForm, RegistrationForm, RequestPasswordResetForm, ResetPasswordForm,
+)
+from warehouse.accounts.interfaces import (
+ InvalidPasswordResetToken, IUserService, IUserTokenService,
+ TooManyFailedLogins,
+)
from warehouse.cache.origin import origin_cache
+from warehouse.email import send_email
from warehouse.packaging.models import Project, Release
from warehouse.utils.http import is_safe_url
@@ -88,7 +95,7 @@ def profile(user, request):
require_methods=False,
)
def login(request, redirect_field_name=REDIRECT_FIELD_NAME,
- _form_class=forms.LoginForm):
+ _form_class=LoginForm):
# TODO: Logging in should reset request.user
# TODO: Configure the login view as the default view for not having
# permission to view something.
@@ -200,7 +207,7 @@ def logout(request, redirect_field_name=REDIRECT_FIELD_NAME):
require_csrf=True,
require_methods=False,
)
-def register(request, _form_class=forms.RegistrationForm):
+def register(request, _form_class=RegistrationForm):
if request.authenticated_userid is not None:
return HTTPSeeOther("/")
@@ -233,6 +240,89 @@ def register(request, _form_class=forms.RegistrationForm):
return {"form": form}
+@view_config(
+ route_name="accounts.request-password-reset",
+ renderer="accounts/request-password-reset.html",
+ uses_session=True,
+ require_csrf=True,
+ require_methods=False,
+)
+def request_password_reset(request, _form_class=RequestPasswordResetForm):
+ user_service = request.find_service(IUserService, context=None)
+ user_token_service = request.find_service(IUserTokenService, context=None)
+ form = _form_class(request.POST, user_service=user_service)
+
+ if request.method == "POST" and form.validate():
+ username = form.username.data
+ user = user_service.get_user_by_username(username)
+ token = user_token_service.generate_token(user)
+ n_hours = user_token_service.token_max_age // 60 // 60
+
+ subject = render(
+ 'email/password-reset.subject.txt',
+ {},
+ request=request,
+ )
+
+ body = render(
+ 'email/password-reset.body.txt',
+ {'token': token, 'username': username, 'n_hours': n_hours},
+ request=request,
+ )
+
+ request.task(send_email).delay(body, [user.email], subject)
+ return {"n_hours": n_hours}
+
+ return {"form": form}
+
+
+@view_config(
+ route_name="accounts.reset-password",
+ renderer="accounts/reset-password.html",
+ uses_session=True,
+ require_csrf=True,
+ require_methods=False,
+)
+def reset_password(request, _form_class=ResetPasswordForm):
+ user_service = request.find_service(IUserService, context=None)
+ user_token_service = request.find_service(IUserTokenService, context=None)
+
+ try:
+ token = request.params.get('token')
+ user = user_token_service.get_user_by_token(token)
+ except InvalidPasswordResetToken as e:
+ # Fail if the token is invalid for any reason
+ request.session.flash(e.message, queue="error")
+ return HTTPSeeOther(
+ request.route_path("accounts.request-password-reset"),
+ )
+
+ form = _form_class(
+ request.params,
+ username=user.username,
+ full_name=user.name,
+ email=user.email,
+ user_service=user_service
+ )
+
+ if request.method == "POST" and form.validate():
+ # Update password.
+ user_service.update_user(user.id, password=form.password.data)
+
+ # Flash a success message
+ request.session.flash(
+ "You have successfully reset your password", queue="success"
+ )
+
+ # Perform login just after reset password and redirect to default view.
+ return HTTPSeeOther(
+ request.route_path("index"),
+ headers=dict(_login_user(request, user.id))
+ )
+
+ return {"form": form}
+
+
@view_config(
route_name="accounts.edit_gravatar",
renderer="accounts/csi/edit_gravatar.csi.html",
diff --git a/warehouse/config.py b/warehouse/config.py
--- a/warehouse/config.py
+++ b/warehouse/config.py
@@ -172,6 +172,18 @@ def configure(settings=None):
maybe_set(settings, "mail.ssl", "MAIL_SSL", default=True)
maybe_set(settings, "ga.tracking_id", "GA_TRACKING_ID")
maybe_set(settings, "statuspage.url", "STATUSPAGE_URL")
+ maybe_set(
+ settings,
+ "password_reset.secret",
+ "PASSWORD_RESET_SECRET_KEY"
+ )
+ maybe_set(
+ settings,
+ "password_reset.token_max_age",
+ "PASSWORD_RESET_TOKEN_MAX_AGE",
+ coercer=int,
+ default=21600, # 6 hours
+ )
maybe_set_compound(settings, "files", "backend", "FILES_BACKEND")
maybe_set_compound(settings, "origin_cache", "backend", "ORIGIN_CACHE")
diff --git a/warehouse/routes.py b/warehouse/routes.py
--- a/warehouse/routes.py
+++ b/warehouse/routes.py
@@ -87,6 +87,16 @@ def includeme(config):
"/account/register/",
domain=warehouse,
)
+ config.add_route(
+ "accounts.request-password-reset",
+ "/account/request-password-reset/",
+ domain=warehouse,
+ )
+ config.add_route(
+ "accounts.reset-password",
+ "/account/reset-password/",
+ domain=warehouse,
+ )
config.add_route(
"accounts.edit_gravatar",
"/user/{username}/edit_gravatar/",
diff --git a/warehouse/utils/crypto.py b/warehouse/utils/crypto.py
--- a/warehouse/utils/crypto.py
+++ b/warehouse/utils/crypto.py
@@ -15,11 +15,16 @@
import os
from itsdangerous import (
- BadSignature, Signer as _Signer, TimestampSigner as _TimestampSigner,
+ BadData, BadSignature, SignatureExpired, Signer as _Signer,
+ TimestampSigner as _TimestampSigner,
+ URLSafeTimedSerializer as _URLSafeTimedSerializer
)
-__all__ = ["BadSignature", "Signer", "TimestampSigner", "random_token"]
+__all__ = [
+ "BadData", "BadSignature", "Signer", "SignatureExpired", "TimestampSigner",
+ "URLSafeTimedSerializer", "random_token"
+]
def random_token():
@@ -37,3 +42,8 @@ class TimestampSigner(_TimestampSigner):
default_digest_method = hashlib.sha512
default_key_derivation = "hmac"
+
+
+class URLSafeTimedSerializer(_URLSafeTimedSerializer):
+
+ default_signer = TimestampSigner
| diff --git a/tests/conftest.py b/tests/conftest.py
--- a/tests/conftest.py
+++ b/tests/conftest.py
@@ -25,6 +25,7 @@
from sqlalchemy import event
from warehouse.config import configure
+from warehouse.accounts import services
from .common.db import Session
@@ -106,6 +107,7 @@ def app_config(database):
"elasticsearch.url": "https://localhost/warehouse",
"files.backend": "warehouse.packaging.services.LocalFileStorage",
"files.url": "http://localhost:7000/",
+ "password_reset.secret": "insecure secret",
"sessions.secret": "123456",
"sessions.url": "redis://localhost:0/",
"statuspage.url": "https://2p66nmmycsj3.statuspage.io",
@@ -144,6 +146,20 @@ def restart_savepoint(session, transaction):
engine.dispose()
[email protected]_fixture
+def user_service(db_session, app_config):
+ return services.DatabaseUserService(
+ db_session, app_config.registry.settings
+ )
+
+
[email protected]_fixture
+def token_service(app_config, user_service):
+ return services.UserTokenService(
+ user_service, app_config.registry.settings
+ )
+
+
class QueryRecorder:
def __init__(self):
diff --git a/tests/unit/accounts/test_forms.py b/tests/unit/accounts/test_forms.py
--- a/tests/unit/accounts/test_forms.py
+++ b/tests/unit/accounts/test_forms.py
@@ -124,6 +124,7 @@ def check_password(userid, password):
class TestRegistrationForm:
+
def test_create(self):
user_service = pretend.stub()
recaptcha_service = pretend.stub(enabled=True)
@@ -350,3 +351,83 @@ def test_password_strength(self):
)
form.validate()
assert (len(form.password.errors) == 0) == valid
+
+
+class TestRequestPasswordResetForm:
+
+ def test_creation(self):
+ user_service = pretend.stub()
+ form = forms.RequestPasswordResetForm(user_service=user_service)
+ assert form.user_service is user_service
+
+ def test_no_password_field(self):
+ user_service = pretend.stub()
+ form = forms.RequestPasswordResetForm(user_service=user_service)
+ assert 'password' not in form._fields
+
+ def test_validate_username_with_no_user(self):
+ user_service = pretend.stub(
+ find_userid=pretend.call_recorder(lambda userid: None),
+ )
+ form = forms.RequestPasswordResetForm(user_service=user_service)
+ field = pretend.stub(data="my_username")
+
+ with pytest.raises(wtforms.validators.ValidationError):
+ form.validate_username(field)
+
+ assert user_service.find_userid.calls == [pretend.call("my_username")]
+
+ def test_validate_username_with_user(self):
+ user_service = pretend.stub(
+ find_userid=pretend.call_recorder(lambda userid: 1),
+ )
+ form = forms.RequestPasswordResetForm(user_service=user_service)
+ field = pretend.stub(data="my_username")
+
+ form.validate_username(field)
+
+ assert user_service.find_userid.calls == [pretend.call("my_username")]
+
+
+class TestResetPasswordForm:
+
+ def test_password_confirm_required_error(self):
+ form = forms.ResetPasswordForm(data={"password_confirm": ""})
+
+ assert not form.validate()
+ assert form.password_confirm.errors.pop() == "This field is required."
+
+ def test_passwords_mismatch_error(self):
+ form = forms.ResetPasswordForm(
+ data={
+ "password": "password",
+ "password_confirm": "mismatch",
+ },
+ )
+
+ assert not form.validate()
+ assert (
+ form.password_confirm.errors.pop() ==
+ "Your passwords do not match. Please try again."
+ )
+
+ @pytest.mark.parametrize(("password", "expected"), [
+ ("foobar", False),
+ ("somethingalittlebetter9", True),
+ ("1aDeCent!1", True),
+ ])
+ def test_password_strength(self, password, expected):
+ form = forms.ResetPasswordForm(
+ data={"password": password, "password_confirm": password},
+ )
+ assert form.validate() == expected
+
+ def test_passwords_match_success(self):
+ form = forms.ResetPasswordForm(
+ data={
+ "password": "MyStr0ng!shPassword",
+ "password_confirm": "MyStr0ng!shPassword",
+ },
+ )
+
+ assert form.validate()
diff --git a/tests/unit/accounts/test_services.py b/tests/unit/accounts/test_services.py
--- a/tests/unit/accounts/test_services.py
+++ b/tests/unit/accounts/test_services.py
@@ -10,15 +10,20 @@
# See the License for the specific language governing permissions and
# limitations under the License.
+import datetime
import uuid
+import freezegun
import pretend
import pytest
from zope.interface.verify import verifyClass
from warehouse.accounts import services
-from warehouse.accounts.interfaces import IUserService, TooManyFailedLogins
+from warehouse.accounts.interfaces import (
+ InvalidPasswordResetToken, IUserService, IUserTokenService,
+ TooManyFailedLogins,
+)
from warehouse.rate_limiting.interfaces import IRateLimiter
from ...common.db.accounts import UserFactory, EmailFactory
@@ -58,158 +63,257 @@ def test_service_creation(self, monkeypatch):
),
]
- def test_find_userid_nonexistant_user(self, db_session):
- service = services.DatabaseUserService(db_session)
- assert service.find_userid("my_username") is None
+ def test_find_userid_nonexistant_user(self, user_service):
+ assert user_service.find_userid("my_username") is None
- def test_find_userid_existing_user(self, db_session):
+ def test_find_userid_existing_user(self, user_service):
user = UserFactory.create()
- service = services.DatabaseUserService(db_session)
- assert service.find_userid(user.username) == user.id
+ assert user_service.find_userid(user.username) == user.id
- def test_check_password_global_rate_limited(self):
+ def test_check_password_global_rate_limited(self, user_service):
resets = pretend.stub()
limiter = pretend.stub(test=lambda: False, resets_in=lambda: resets)
- service = services.DatabaseUserService(
- pretend.stub(),
- ratelimiters={"global": limiter},
- )
+ user_service.ratelimiters["global"] = limiter
with pytest.raises(TooManyFailedLogins) as excinfo:
- service.check_password(uuid.uuid4(), None)
+ user_service.check_password(uuid.uuid4(), None)
assert excinfo.value.resets_in is resets
- def test_check_password_nonexistant_user(self, db_session):
- service = services.DatabaseUserService(db_session)
- assert not service.check_password(uuid.uuid4(), None)
+ def test_check_password_nonexistant_user(self, user_service):
+ assert not user_service.check_password(uuid.uuid4(), None)
- def test_check_password_user_rate_limited(self, db_session):
+ def test_check_password_user_rate_limited(self, user_service):
user = UserFactory.create()
resets = pretend.stub()
limiter = pretend.stub(
test=pretend.call_recorder(lambda uid: False),
resets_in=pretend.call_recorder(lambda uid: resets),
)
- service = services.DatabaseUserService(
- db_session,
- ratelimiters={"user": limiter},
- )
+ user_service.ratelimiters["user"] = limiter
with pytest.raises(TooManyFailedLogins) as excinfo:
- service.check_password(user.id, None)
+ user_service.check_password(user.id, None)
assert excinfo.value.resets_in is resets
assert limiter.test.calls == [pretend.call(user.id)]
assert limiter.resets_in.calls == [pretend.call(user.id)]
- def test_check_password_invalid(self, db_session):
+ def test_check_password_invalid(self, user_service):
user = UserFactory.create()
- service = services.DatabaseUserService(db_session)
- service.hasher = pretend.stub(
+ user_service.hasher = pretend.stub(
verify_and_update=pretend.call_recorder(
lambda l, r: (False, None)
),
)
- assert not service.check_password(user.id, "user password")
- assert service.hasher.verify_and_update.calls == [
+ assert not user_service.check_password(user.id, "user password")
+ assert user_service.hasher.verify_and_update.calls == [
pretend.call("user password", user.password),
]
- def test_check_password_valid(self, db_session):
+ def test_check_password_valid(self, user_service):
user = UserFactory.create()
- service = services.DatabaseUserService(db_session)
- service.hasher = pretend.stub(
+ user_service.hasher = pretend.stub(
verify_and_update=pretend.call_recorder(lambda l, r: (True, None)),
)
- assert service.check_password(user.id, "user password")
- assert service.hasher.verify_and_update.calls == [
+ assert user_service.check_password(user.id, "user password")
+ assert user_service.hasher.verify_and_update.calls == [
pretend.call("user password", user.password),
]
- def test_check_password_updates(self, db_session):
+ def test_check_password_updates(self, user_service):
user = UserFactory.create()
password = user.password
- service = services.DatabaseUserService(db_session)
- service.hasher = pretend.stub(
+ user_service.hasher = pretend.stub(
verify_and_update=pretend.call_recorder(
lambda l, r: (True, "new password")
),
)
- assert service.check_password(user.id, "user password")
- assert service.hasher.verify_and_update.calls == [
+ assert user_service.check_password(user.id, "user password")
+ assert user_service.hasher.verify_and_update.calls == [
pretend.call("user password", password),
]
assert user.password == "new password"
- def test_create_user(self, db_session):
+ def test_create_user(self, user_service):
user = UserFactory.build()
email = "[email protected]"
- service = services.DatabaseUserService(db_session)
- new_user = service.create_user(username=user.username,
- name=user.name,
- password=user.password,
- email=email)
- db_session.flush()
- user_from_db = service.get_user(new_user.id)
+ new_user = user_service.create_user(
+ username=user.username,
+ name=user.name,
+ password=user.password,
+ email=email
+ )
+ user_service.db.flush()
+ user_from_db = user_service.get_user(new_user.id)
assert user_from_db.username == user.username
assert user_from_db.name == user.name
assert user_from_db.email == email
- def test_update_user(self, db_session):
+ def test_update_user(self, user_service):
user = UserFactory.create()
- service = services.DatabaseUserService(db_session)
new_name, password = "new username", "TestPa@@w0rd"
- service.update_user(user.id, username=new_name, password=password)
- user_from_db = service.get_user(user.id)
+ user_service.update_user(user.id, username=new_name, password=password)
+ user_from_db = user_service.get_user(user.id)
assert user_from_db.username == user.username
assert password != user_from_db.password
- assert service.hasher.verify(password, user_from_db.password)
+ assert user_service.hasher.verify(password, user_from_db.password)
- def test_verify_email(self, db_session):
- service = services.DatabaseUserService(db_session)
+ def test_verify_email(self, user_service):
user = UserFactory.create()
EmailFactory.create(user=user, primary=True,
verified=False)
EmailFactory.create(user=user, primary=False,
verified=False)
- service.verify_email(user.id, user.emails[0].email)
+ user_service.verify_email(user.id, user.emails[0].email)
assert user.emails[0].verified
assert not user.emails[1].verified
- def test_find_by_email(self, db_session):
- service = services.DatabaseUserService(db_session)
+ def test_find_by_email(self, user_service):
user = UserFactory.create()
EmailFactory.create(user=user, primary=True, verified=False)
- found_userid = service.find_userid_by_email(user.emails[0].email)
- db_session.flush()
+ found_userid = user_service.find_userid_by_email(user.emails[0].email)
+ user_service.db.flush()
assert user.id == found_userid
- def test_find_by_email_not_found(self, db_session):
- service = services.DatabaseUserService(db_session)
- assert service.find_userid_by_email("something") is None
+ def test_find_by_email_not_found(self, user_service):
+ assert user_service.find_userid_by_email("something") is None
- def test_create_login_success(self, db_session):
- service = services.DatabaseUserService(db_session)
- user = service.create_user(
+ def test_create_login_success(self, user_service):
+ user = user_service.create_user(
"test_user", "test_name", "test_password", "test_email")
assert user.id is not None
# now make sure that we can log in as that user
- assert service.check_password(user.id, "test_password")
+ assert user_service.check_password(user.id, "test_password")
- def test_create_login_error(self, db_session):
- service = services.DatabaseUserService(db_session)
- user = service.create_user(
+ def test_create_login_error(self, user_service):
+ user = user_service.create_user(
"test_user", "test_name", "test_password", "test_email")
assert user.id is not None
- assert not service.check_password(user.id, "bad_password")
+ assert not user_service.check_password(user.id, "bad_password")
+
+ def test_get_user_by_username(self, user_service):
+ user = UserFactory.create()
+ found_user = user_service.get_user_by_username(user.username)
+ user_service.db.flush()
+
+ assert user.username == found_user.username
+
+ def test_get_user_by_username_failure(self, user_service):
+ UserFactory.create()
+ found_user = user_service.get_user_by_username("UNKNOWNTOTHEWORLD")
+ user_service.db.flush()
+
+ assert found_user is None
+
+
+class TestUserTokenService:
+
+ def test_verify_service(self):
+ assert verifyClass(IUserTokenService, services.UserTokenService)
+
+ def test_service_creation(self, monkeypatch):
+ serializer_obj = pretend.stub()
+ serializer_cls = pretend.call_recorder(lambda *a, **kw: serializer_obj)
+ monkeypatch.setattr(services, "URLSafeTimedSerializer", serializer_cls)
+
+ secret = pretend.stub()
+ max_age = pretend.stub()
+ settings = {
+ "password_reset.secret": secret,
+ "password_reset.token_max_age": max_age,
+ }
+ user_service = pretend.stub()
+ service = services.UserTokenService(user_service, settings)
+
+ assert service.serializer == serializer_obj
+ assert service.token_max_age == max_age
+ assert serializer_cls.calls == [
+ pretend.call(secret, salt="password-reset")
+ ]
+
+ def test_generate_token(self, token_service):
+ user = UserFactory.create()
+ assert token_service.generate_token(user)
+
+ @pytest.mark.parametrize('token', ['', None])
+ def test_get_user_by_token_is_none(self, token_service, token):
+ with pytest.raises(InvalidPasswordResetToken) as e:
+ token_service.get_user_by_token(token)
+ assert e.value.message == "Invalid token - No token supplied"
+
+ def test_get_user_by_token_invalid(self, token_service):
+ with pytest.raises(InvalidPasswordResetToken) as e:
+ token_service.get_user_by_token("definitely not valid")
+ assert e.value.message == (
+ "Invalid token - Request a new password reset link"
+ )
+
+ def test_get_user_by_token_is_expired(self, token_service):
+ user = UserFactory.create()
+ now = datetime.datetime.utcnow()
+
+ with freezegun.freeze_time(now) as frozen_time:
+ token = token_service.generate_token(user)
+
+ frozen_time.tick(
+ delta=datetime.timedelta(
+ seconds=token_service.token_max_age + 1,
+ )
+ )
+
+ with pytest.raises(InvalidPasswordResetToken) as e:
+ token_service.get_user_by_token(token)
+ assert e.value.message == (
+ "Expired token - Token is expired, request a new password "
+ "reset link"
+ )
+
+ def test_get_user_by_token_invalid_user(self, token_service):
+ invalid_user = pretend.stub(
+ id="8ad1a4ac-e016-11e6-bf01-fe55135034f3",
+ last_login="lastlogintimestamp",
+ password_date="passworddate"
+ )
+
+ token = token_service.generate_token(invalid_user)
+ with pytest.raises(InvalidPasswordResetToken) as e:
+ token_service.get_user_by_token(token)
+ assert e.value.message == "Invalid token - User not found"
+
+ def test_get_user_by_token_last_login_changed(self, token_service):
+ user = UserFactory.create()
+ token = token_service.generate_token(user)
+ user.last_login = datetime.datetime.now()
+ with pytest.raises(InvalidPasswordResetToken) as e:
+ token_service.get_user_by_token(token)
+ assert e.value.message == (
+ "Invalid token - User has logged in since this token was requested"
+ )
+
+ def test_get_user_by_token_password_date_changed(self, token_service):
+ user = UserFactory.create()
+ token = token_service.generate_token(user)
+ user.password_date = datetime.datetime.now()
+ with pytest.raises(InvalidPasswordResetToken) as e:
+ token_service.get_user_by_token(token)
+ assert e.value.message == (
+ "Invalid token - Password has already been changed since this "
+ "token was requested"
+ )
+
+ def test_get_user_by_token_success(self, token_service):
+ user = UserFactory.create()
+ token = token_service.generate_token(user)
+
+ assert token_service.get_user_by_token(token) == user
def test_database_login_factory(monkeypatch):
@@ -248,3 +352,25 @@ def find_service(iface, name, context):
},
),
]
+
+
+def test_user_token_factory(monkeypatch):
+ service_obj = pretend.stub()
+ service_cls = pretend.call_recorder(lambda session, settings: service_obj)
+ monkeypatch.setattr(services, "UserTokenService", service_cls)
+ user_service = pretend.stub()
+
+ def find_service(iface):
+ assert iface is IUserService
+ return user_service
+
+ context = pretend.stub()
+ request = pretend.stub(
+ find_service=find_service,
+ registry=pretend.stub(settings=pretend.stub())
+ )
+
+ assert services.user_token_factory(context, request) is service_obj
+ assert service_cls.calls == [
+ pretend.call(user_service, request.registry.settings),
+ ]
diff --git a/tests/unit/accounts/test_views.py b/tests/unit/accounts/test_views.py
--- a/tests/unit/accounts/test_views.py
+++ b/tests/unit/accounts/test_views.py
@@ -20,7 +20,10 @@
from pyramid.httpexceptions import HTTPMovedPermanently, HTTPSeeOther
from warehouse.accounts import views
-from warehouse.accounts.interfaces import IUserService, TooManyFailedLogins
+from warehouse.accounts.interfaces import (
+ IUserService, IUserTokenService, TooManyFailedLogins
+)
+from warehouse.accounts.services import InvalidPasswordResetToken
from ...common.db.accounts import UserFactory
@@ -276,6 +279,7 @@ def test_post_redirects_user(self, pyramid_request, expected_next_url,
class TestRegister:
+
def test_get(self, pyramid_request):
form_inst = pretend.stub()
form = pretend.call_recorder(lambda *args, **kwargs: form_inst)
@@ -324,6 +328,237 @@ def test_register_redirect(self, pyramid_request):
assert result.headers["Location"] == "/"
+class TestRequestPasswordReset:
+
+ def test_get(self, pyramid_request, user_service):
+ form_inst = pretend.stub()
+ form_class = pretend.call_recorder(lambda *args, **kwargs: form_inst)
+ pyramid_request.find_service = pretend.call_recorder(
+ lambda *args, **kwargs: user_service
+ )
+ pyramid_request.POST = pretend.stub()
+ result = views.request_password_reset(
+ pyramid_request,
+ _form_class=form_class,
+ )
+ assert result["form"] is form_inst
+ assert form_class.calls == [
+ pretend.call(pyramid_request.POST, user_service=user_service),
+ ]
+ assert pyramid_request.find_service.calls == [
+ pretend.call(IUserService, context=None),
+ pretend.call(IUserTokenService, context=None),
+ ]
+
+ def test_request_password_reset(
+ self, monkeypatch, pyramid_request, pyramid_config, user_service,
+ token_service):
+
+ stub_user = pretend.stub(email="email", username="username_value")
+ pyramid_request.method = "POST"
+ token_service.generate_token = pretend.call_recorder(lambda a: "TOK")
+ user_service.get_user_by_username = pretend.call_recorder(
+ lambda a: stub_user
+ )
+ pyramid_request.find_service = pretend.call_recorder(
+ lambda interface, **kwargs: {
+ IUserService: user_service,
+ IUserTokenService: token_service,
+ }[interface]
+ )
+ pyramid_request.POST = {"username": stub_user.username}
+
+ subject_renderer = pyramid_config.testing_add_renderer(
+ 'email/password-reset.subject.txt'
+ )
+ subject_renderer.string_response = 'Email Subject'
+ body_renderer = pyramid_config.testing_add_renderer(
+ 'email/password-reset.body.txt'
+ )
+ body_renderer.string_response = 'Email Body'
+
+ form_obj = pretend.stub(
+ username=pretend.stub(data=stub_user.username),
+ validate=pretend.call_recorder(lambda: True),
+ )
+ form_class = pretend.call_recorder(lambda d, user_service: form_obj)
+ send_email = pretend.stub(
+ delay=pretend.call_recorder(lambda *args, **kwargs: None)
+ )
+ pyramid_request.task = pretend.call_recorder(
+ lambda *args, **kwargs: send_email
+ )
+ monkeypatch.setattr(views, "send_email", send_email)
+
+ result = views.request_password_reset(
+ pyramid_request, _form_class=form_class
+ )
+
+ assert result == {"n_hours": token_service.token_max_age // 60 // 60}
+ subject_renderer.assert_()
+ body_renderer.assert_(token="TOK", username=stub_user.username)
+ assert token_service.generate_token.calls == [
+ pretend.call(stub_user),
+ ]
+ assert user_service.get_user_by_username.calls == [
+ pretend.call(stub_user.username),
+ ]
+ assert pyramid_request.find_service.calls == [
+ pretend.call(IUserService, context=None),
+ pretend.call(IUserTokenService, context=None),
+ ]
+ assert form_obj.validate.calls == [
+ pretend.call(),
+ ]
+ assert form_class.calls == [
+ pretend.call(pyramid_request.POST, user_service=user_service),
+ ]
+ assert pyramid_request.task.calls == [
+ pretend.call(send_email),
+ ]
+ assert send_email.delay.calls == [
+ pretend.call('Email Body', [stub_user.email], 'Email Subject'),
+ ]
+
+
+class TestResetPassword:
+
+ def test_invalid_token(self, pyramid_request, user_service, token_service):
+ form_inst = pretend.stub()
+ form_class = pretend.call_recorder(lambda *args, **kwargs: form_inst)
+
+ def get_user_by_token(token):
+ raise InvalidPasswordResetToken('message')
+
+ pyramid_request.GET.update({"token": "RANDOM_KEY"})
+ token_service.get_user_by_token = pretend.call_recorder(
+ get_user_by_token
+ )
+ pyramid_request.find_service = pretend.call_recorder(
+ lambda interface, **kwargs: {
+ IUserService: user_service,
+ IUserTokenService: token_service,
+ }[interface]
+ )
+ pyramid_request.route_path = pretend.call_recorder(lambda name: "/")
+ pyramid_request.session.flash = pretend.call_recorder(
+ lambda *a, **kw: None
+ )
+
+ views.reset_password(pyramid_request, _form_class=form_class)
+
+ assert form_class.calls == []
+ assert pyramid_request.find_service.calls == [
+ pretend.call(IUserService, context=None),
+ pretend.call(IUserTokenService, context=None),
+ ]
+ assert token_service.get_user_by_token.calls == [
+ pretend.call("RANDOM_KEY"),
+ ]
+ assert pyramid_request.route_path.calls == [
+ pretend.call('accounts.request-password-reset'),
+ ]
+ assert pyramid_request.session.flash.calls == [
+ pretend.call('message', queue='error'),
+ ]
+
+ def test_get(self, db_request, user_service, token_service):
+ user = UserFactory.create()
+ form_inst = pretend.stub()
+ form_class = pretend.call_recorder(lambda *args, **kwargs: form_inst)
+
+ db_request.GET.update({"token": "RANDOM_KEY"})
+ token_service.get_user_by_token = pretend.call_recorder(
+ lambda token: user
+ )
+ db_request.find_service = pretend.call_recorder(
+ lambda interface, **kwargs: {
+ IUserService: user_service,
+ IUserTokenService: token_service,
+ }[interface]
+ )
+
+ result = views.reset_password(db_request, _form_class=form_class)
+
+ assert result["form"] is form_inst
+ assert form_class.calls == [
+ pretend.call(
+ db_request.GET,
+ username=user.username,
+ full_name=user.name,
+ email=user.email,
+ user_service=user_service,
+ )
+ ]
+ assert token_service.get_user_by_token.calls == [
+ pretend.call("RANDOM_KEY"),
+ ]
+ assert db_request.find_service.calls == [
+ pretend.call(IUserService, context=None),
+ pretend.call(IUserTokenService, context=None),
+ ]
+
+ def test_reset_password(self, db_request, user_service, token_service):
+ user = UserFactory.create()
+ db_request.method = "POST"
+ db_request.POST.update({"token": "RANDOM_KEY"})
+ form_obj = pretend.stub(
+ password=pretend.stub(data="password_value"),
+ validate=pretend.call_recorder(lambda *args: True)
+ )
+
+ form_class = pretend.call_recorder(lambda *args, **kwargs: form_obj)
+
+ db_request.route_path = pretend.call_recorder(lambda name: "/")
+ token_service.get_user_by_token = pretend.call_recorder(lambda a: user)
+ user_service.update_user = pretend.call_recorder(lambda *a, **kw: None)
+ db_request.find_service = pretend.call_recorder(
+ lambda interface, **kwargs: {
+ IUserService: user_service,
+ IUserTokenService: token_service,
+ }[interface]
+ )
+ db_request.session.flash = pretend.call_recorder(
+ lambda *a, **kw: None
+ )
+
+ now = datetime.datetime.utcnow()
+
+ with freezegun.freeze_time(now):
+ result = views.reset_password(db_request, _form_class=form_class)
+
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/"
+ assert form_obj.validate.calls == [pretend.call()]
+ assert form_class.calls == [
+ pretend.call(
+ db_request.POST,
+ username=user.username,
+ full_name=user.name,
+ email=user.email,
+ user_service=user_service
+ ),
+ ]
+ assert db_request.route_path.calls == [pretend.call('index')]
+ assert token_service.get_user_by_token.calls == [
+ pretend.call('RANDOM_KEY'),
+ ]
+ assert user_service.update_user.calls == [
+ pretend.call(user.id, password=form_obj.password.data),
+ pretend.call(user.id, last_login=now),
+ ]
+ assert db_request.session.flash.calls == [
+ pretend.call(
+ "You have successfully reset your password", queue="success"
+ ),
+ ]
+ assert db_request.find_service.calls == [
+ pretend.call(IUserService, context=None),
+ pretend.call(IUserTokenService, context=None),
+ pretend.call(IUserService, context=None),
+ ]
+
+
class TestClientSideIncludes:
def test_edit_gravatar_csi_returns_user(self, db_request):
diff --git a/tests/unit/test_config.py b/tests/unit/test_config.py
--- a/tests/unit/test_config.py
+++ b/tests/unit/test_config.py
@@ -255,7 +255,8 @@ def __init__(self):
"warehouse.env": environment,
"warehouse.commit": None,
"site.name": "Warehouse",
- "mail.ssl": True
+ "mail.ssl": True,
+ 'password_reset.token_max_age': 21600,
}
if environment == config.Environment.development:
diff --git a/tests/unit/test_routes.py b/tests/unit/test_routes.py
--- a/tests/unit/test_routes.py
+++ b/tests/unit/test_routes.py
@@ -115,6 +115,16 @@ def add_policy(name, filename):
"/account/register/",
domain=warehouse,
),
+ pretend.call(
+ "accounts.request-password-reset",
+ "/account/request-password-reset/",
+ domain=warehouse,
+ ),
+ pretend.call(
+ "accounts.reset-password",
+ "/account/reset-password/",
+ domain=warehouse,
+ ),
pretend.call(
"accounts.edit_gravatar",
"/user/{username}/edit_gravatar/",
| Implement "forgot password" feature
- A new page that will be linked to from the sign-in and register page.
- Asks for username
- Email the user with a temporary link to change the password
| I've added this to the launch milestone because it would be really nice to get this working soon :)
@dstufft @nlhkabu @karan Anyone started working on this? If not, can I implement this?
As far as I know nobody has started working on it, so feel free :)
@gsb-eng I have not. I'll be out of town without a computer so please go for it. :)
I'm working on this.
+1
Need this feature, when I try to register an account with my primary email, it says an account with the email already exists, but I don't remeber the password
+1 me too. I cannot publish my packages any more since it seems like https://pypi.python.org won't accept new uploads.
Is there a workaround? I can log into https://pypi.python.org/ just fine.
One work-around I have discovered for anyone else with this problem:
- Create new user on pypi.python.org
- Assign new user as additional owner of project you want to update
- Create .pypirc entry with new user's credentials and pypi.org as repository
In our [meeting today](https://wiki.python.org/psf/PackagingWG/2017-12-19-Warehouse) we decided this belongs in [the first milestone, the MVP for package maintainers](https://github.com/pypa/warehouse/milestone/8). | 2018-01-10T20:45:30Z | [] | [] |
pypi/warehouse | 2,765 | pypi__warehouse-2765 | [
"2486"
] | 2044fcb81a5a33c87daf920a70f9c3952e833e61 | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -875,7 +875,13 @@ def file_upload(request):
lambda: request.POST["content"].file.read(8096), b""):
file_size += len(chunk)
if file_size > file_size_limit:
- raise _exc_with_message(HTTPBadRequest, "File too large.")
+ raise _exc_with_message(
+ HTTPBadRequest,
+ "File too large. " +
+ "Limit for project {name!r} is {limit}MB".format(
+ name=project.name,
+ limit=file_size_limit // (1024 * 1024),
+ ))
fp.write(chunk)
for hasher in file_hashes.values():
hasher.update(chunk)
| diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -1371,7 +1371,10 @@ def test_upload_fails_with_too_large_file(self, pyramid_config,
pyramid_config.testing_securitypolicy(userid=1)
user = UserFactory.create()
- project = ProjectFactory.create()
+ project = ProjectFactory.create(
+ name='foobar',
+ upload_limit=(60 * 1024 * 1024), # 60MB
+ )
release = ReleaseFactory.create(project=project, version="1.0")
RoleFactory.create(user=user, project=project)
@@ -1385,7 +1388,7 @@ def test_upload_fails_with_too_large_file(self, pyramid_config,
"md5_digest": "nope!",
"content": pretend.stub(
filename=filename,
- file=io.BytesIO(b"a" * (legacy.MAX_FILESIZE + 1)),
+ file=io.BytesIO(b"a" * (project.upload_limit + 1)),
type="application/tar",
),
})
@@ -1396,7 +1399,9 @@ def test_upload_fails_with_too_large_file(self, pyramid_config,
resp = excinfo.value
assert resp.status_code == 400
- assert resp.status == "400 File too large."
+ assert resp.status == (
+ "400 File too large. Limit for project 'foobar' is 60MB"
+ )
def test_upload_fails_with_too_large_signature(self, pyramid_config,
db_request):
| File size limit error message should include size of the limit
Since we don't document the file size limit for uploads anywhere, it'd be nice if the error message when an upload is over the limit includes what the limit is, especially considering that the limits might be different for various projects.
Currently the message is something like:
```
HTTPError: 400 Client Error: File too large. for url: https://upload.pypi.org/legacy/
```
It'd be nice if this was something like:
```
HTTPError: 400 Client Error: File too large (limit for <project_name> is <limit>) for url: https://upload.pypi.org/legacy/
```
| In our [meeting today](https://wiki.python.org/psf/PackagingWG/2017-12-19-Warehouse) we decided this belongs in [the first milestone, the MVP for package maintainers](https://github.com/pypa/warehouse/milestone/8).
@Miserlou is working on implementing it in #2487.
I closed #2487, so this issue is still open and available for someone else to pick up as their first contribution.
@di
https://github.com/pypa/warehouse/blob/d12b7c1ff792942b6ba8c91793e4fa6e1f1cf137/warehouse/forklift/legacy.py#L878
It's just the matter of formatting the project name and file size limit from local variables here into the message; correct?
@pradyunsg Yep! Something like:
> File is too large. Limit for project 'foobar' is 60MB. | 2018-01-11T16:29:08Z | [] | [] |
pypi/warehouse | 2,767 | pypi__warehouse-2767 | [
"2668"
] | 5717e3c968df86e384504424f60f85a971ee1b05 | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -274,6 +274,7 @@ class MetadataForm(forms.Form):
# Metadata version
metadata_version = wtforms.StringField(
+ description="Metadata-Version",
validators=[
wtforms.validators.DataRequired(),
wtforms.validators.AnyOf(
@@ -288,6 +289,7 @@ class MetadataForm(forms.Form):
# Identity Project and Release
name = wtforms.StringField(
+ description="Name",
validators=[
wtforms.validators.DataRequired(),
wtforms.validators.Regexp(
@@ -301,6 +303,7 @@ class MetadataForm(forms.Form):
],
)
version = wtforms.StringField(
+ description="Version",
validators=[
wtforms.validators.DataRequired(),
wtforms.validators.Regexp(
@@ -313,6 +316,7 @@ class MetadataForm(forms.Form):
# Additional Release metadata
summary = wtforms.StringField(
+ description="Summary",
validators=[
wtforms.validators.Optional(),
wtforms.validators.Length(max=512),
@@ -323,37 +327,57 @@ class MetadataForm(forms.Form):
],
)
description = wtforms.StringField(
+ description="Description",
+ validators=[wtforms.validators.Optional()],
+ )
+ author = wtforms.StringField(
+ description="Author",
validators=[wtforms.validators.Optional()],
)
- author = wtforms.StringField(validators=[wtforms.validators.Optional()])
author_email = wtforms.StringField(
+ description="Author-email",
validators=[
wtforms.validators.Optional(),
wtforms.validators.Email(),
],
)
maintainer = wtforms.StringField(
+ description="Maintainer",
validators=[wtforms.validators.Optional()],
)
maintainer_email = wtforms.StringField(
+ description="Maintainer-email",
validators=[
wtforms.validators.Optional(),
wtforms.validators.Email(),
],
)
- license = wtforms.StringField(validators=[wtforms.validators.Optional()])
- keywords = wtforms.StringField(validators=[wtforms.validators.Optional()])
- classifiers = wtforms.fields.SelectMultipleField()
- platform = wtforms.StringField(validators=[wtforms.validators.Optional()])
+ license = wtforms.StringField(
+ description="License",
+ validators=[wtforms.validators.Optional()],
+ )
+ keywords = wtforms.StringField(
+ description="Keywords",
+ validators=[wtforms.validators.Optional()],
+ )
+ classifiers = wtforms.fields.SelectMultipleField(
+ description="Classifier",
+ )
+ platform = wtforms.StringField(
+ description="Platform",
+ validators=[wtforms.validators.Optional()],
+ )
# URLs
home_page = wtforms.StringField(
+ description="Home-Page",
validators=[
wtforms.validators.Optional(),
forms.URIValidator(),
],
)
download_url = wtforms.StringField(
+ description="Download-URL",
validators=[
wtforms.validators.Optional(),
forms.URIValidator(),
@@ -362,6 +386,7 @@ class MetadataForm(forms.Form):
# Dependency Information
requires_python = wtforms.StringField(
+ description="Requires-Python",
validators=[
wtforms.validators.Optional(),
_validate_pep440_specifier_field,
@@ -384,7 +409,9 @@ class MetadataForm(forms.Form):
),
]
)
- comment = wtforms.StringField(validators=[wtforms.validators.Optional()])
+ comment = wtforms.StringField(
+ validators=[wtforms.validators.Optional()],
+ )
md5_digest = wtforms.StringField(
validators=[
wtforms.validators.Optional(),
@@ -398,7 +425,7 @@ class MetadataForm(forms.Form):
re.IGNORECASE,
message="Must be a valid, hex encoded, SHA256 message digest.",
),
- ]
+ ],
)
blake2_256_digest = wtforms.StringField(
validators=[
@@ -408,7 +435,7 @@ class MetadataForm(forms.Form):
re.IGNORECASE,
message="Must be a valid, hex encoded, blake2 message digest.",
),
- ]
+ ],
)
# Legacy dependency information
@@ -416,7 +443,7 @@ class MetadataForm(forms.Form):
validators=[
wtforms.validators.Optional(),
_validate_legacy_non_dist_req_list,
- ]
+ ],
)
provides = ListField(
validators=[
@@ -433,24 +460,28 @@ class MetadataForm(forms.Form):
# Newer dependency information
requires_dist = ListField(
+ description="Requires-Dist",
validators=[
wtforms.validators.Optional(),
_validate_legacy_dist_req_list,
],
)
provides_dist = ListField(
+ description="Provides-Dist",
validators=[
wtforms.validators.Optional(),
_validate_legacy_dist_req_list,
],
)
obsoletes_dist = ListField(
+ description="Obsoletes-Dist",
validators=[
wtforms.validators.Optional(),
_validate_legacy_dist_req_list,
],
)
requires_external = ListField(
+ description="Requires-External",
validators=[
wtforms.validators.Optional(),
_validate_requires_external_list,
@@ -459,6 +490,7 @@ class MetadataForm(forms.Form):
# Newer metadata information
project_urls = ListField(
+ description="Project-URL",
validators=[
wtforms.validators.Optional(),
_validate_project_url_list,
@@ -649,12 +681,27 @@ def file_upload(request):
else:
field_name = sorted(form.errors.keys())[0]
+ if field_name in form:
+ if form[field_name].description:
+ error_message = (
+ "{value!r} is an invalid value for {field}. ".format(
+ value=form[field_name].data,
+ field=form[field_name].description) +
+ "Error: {} ".format(form.errors[field_name][0]) +
+ "see "
+ "https://packaging.python.org/specifications/core-metadata"
+ )
+ else:
+ error_message = "{field}: {msgs[0]}".format(
+ field=field_name,
+ msgs=form.errors[field_name],
+ )
+ else:
+ error_message = "Error: {}".format(form.errors[field_name][0])
+
raise _exc_with_message(
HTTPBadRequest,
- "{field}: {msgs[0]}".format(
- field=field_name,
- msgs=form.errors[field_name],
- ),
+ error_message,
)
# Ensure that we have file data in the request.
| diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -647,24 +647,45 @@ def test_fails_invalid_version(self, pyramid_config, pyramid_request,
("post_data", "message"),
[
# metadata_version errors.
- ({}, "metadata_version: This field is required."),
+ (
+ {},
+ "None is an invalid value for Metadata-Version. "
+ "Error: This field is required. "
+ "see "
+ "https://packaging.python.org/specifications/core-metadata",
+ ),
(
{"metadata_version": "-1"},
- "metadata_version: Unknown Metadata Version",
+ "'-1' is an invalid value for Metadata-Version. "
+ "Error: Unknown Metadata Version "
+ "see "
+ "https://packaging.python.org/specifications/core-metadata",
),
# name errors.
- ({"metadata_version": "1.2"}, "name: This field is required."),
+ (
+ {"metadata_version": "1.2"},
+ "'' is an invalid value for Name. "
+ "Error: This field is required. "
+ "see "
+ "https://packaging.python.org/specifications/core-metadata",
+ ),
(
{"metadata_version": "1.2", "name": "foo-"},
- "name: Must start and end with a letter or numeral and "
- "contain only ascii numeric and '.', '_' and '-'.",
+ "'foo-' is an invalid value for Name. "
+ "Error: Must start and end with a letter or numeral and "
+ "contain only ascii numeric and '.', '_' and '-'. "
+ "see "
+ "https://packaging.python.org/specifications/core-metadata",
),
# version errors.
(
{"metadata_version": "1.2", "name": "example"},
- "version: This field is required.",
+ "'' is an invalid value for Version. "
+ "Error: This field is required. "
+ "see "
+ "https://packaging.python.org/specifications/core-metadata",
),
(
{
@@ -672,8 +693,11 @@ def test_fails_invalid_version(self, pyramid_config, pyramid_request,
"name": "example",
"version": "dog",
},
- "version: Must start and end with a letter or numeral and "
- "contain only ascii numeric and '.', '_' and '-'.",
+ "'dog' is an invalid value for Version. "
+ "Error: Must start and end with a letter or numeral and "
+ "contain only ascii numeric and '.', '_' and '-'. "
+ "see "
+ "https://packaging.python.org/specifications/core-metadata",
),
# filetype/pyversion errors.
@@ -693,8 +717,8 @@ def test_fails_invalid_version(self, pyramid_config, pyramid_request,
"version": "1.0",
"filetype": "bdist_wat",
},
- "__all__: Python version is required for binary distribution "
- "uploads.",
+ "Error: Python version is required for binary distribution "
+ "uploads."
),
(
{
@@ -715,8 +739,8 @@ def test_fails_invalid_version(self, pyramid_config, pyramid_request,
"filetype": "sdist",
"pyversion": "1.0",
},
- "__all__: The only valid Python version for a sdist is "
- "'source'.",
+ "Error: The only valid Python version for a sdist is "
+ "'source'."
),
# digest errors.
@@ -727,7 +751,18 @@ def test_fails_invalid_version(self, pyramid_config, pyramid_request,
"version": "1.0",
"filetype": "sdist",
},
- "__all__: Must include at least one message digest.",
+ "Error: Must include at least one message digest."
+ ),
+ (
+ {
+ "metadata_version": "1.2",
+ "name": "example",
+ "version": "1.0",
+ "filetype": "sdist",
+ "sha256_digest": "an invalid sha256 digest",
+ },
+ "sha256_digest: "
+ "Must be a valid, hex encoded, SHA256 message digest."
),
# summary errors
@@ -740,7 +775,10 @@ def test_fails_invalid_version(self, pyramid_config, pyramid_request,
"md5_digest": "a fake md5 digest",
"summary": "A" * 513,
},
- "summary: Field cannot be longer than 512 characters.",
+ "'" + "A" * 513 + "' is an invalid value for Summary. "
+ "Error: Field cannot be longer than 512 characters. "
+ "see "
+ "https://packaging.python.org/specifications/core-metadata",
),
(
{
@@ -751,7 +789,10 @@ def test_fails_invalid_version(self, pyramid_config, pyramid_request,
"md5_digest": "a fake md5 digest",
"summary": "A\nB",
},
- "summary: Multiple lines are not allowed.",
+ ("{!r} is an invalid value for Summary. ".format('A\nB') +
+ "Error: Multiple lines are not allowed. "
+ "see "
+ "https://packaging.python.org/specifications/core-metadata"),
),
# classifiers are a FieldStorage
@@ -1263,8 +1304,11 @@ def test_upload_fails_with_invalid_classifier(self, pyramid_config,
assert resp.status_code == 400
assert resp.status == (
- "400 classifiers: 'Environment :: Other Environment' is not a "
- "valid choice for this field"
+ "400 ['Environment :: Other Environment'] "
+ "is an invalid value for Classifier. "
+ "Error: 'Environment :: Other Environment' is not a valid choice "
+ "for this field "
+ "see https://packaging.python.org/specifications/core-metadata"
)
@pytest.mark.parametrize(
| Better error messages when metadata does not validate
Currently, if a kwarg in `setup.py` does not pass validation, the response from Warehouse is not really descriptive enough to indicate what the issue is.
For example, if one has an invalid `url`:
```
url='foobar',
```
The response looks like:
```
HTTPError: 400 Client Error: home_page: Invalid URI for url: http://pypi.org/legacy/
```
This message is confusing because:
* it refers to both `home_page` and `url`
* the former of which is what the actual metadata field is called (but even still it's `Home-Page` in the specifications
* the latter of which is what setuptools calls it;
* the error message from twine includes the repo url, but the issue is with the kwarg value (`foobar`)
A better error message might be something like:
```
HTTPError: 400 Client Error: "foobar" is an invalid value for field "Home-Page",
see https://packaging.python.org/specifications/core-metadata/
```
But the ambiguity between `url` and `home-page` remains.
| Where is this validation taking place? After some searching I couldn't find the place where the error was being generated. Closes thing I found was this snippet in `forklift/legacy.py`
``` python
if not form.validate():
for field_name in _error_message_order:
if field_name in form.errors:
break
else:
field_name = sorted(form.errors.keys())[0]
raise _exc_with_message(
HTTPBadRequest,
"{field}: {msgs[0]}".format(
field=field_name,
msgs=form.errors[field_name],
),
)
```
@alanbato Great question. It's a bit confusing, only a part of the error message that comes from Warehouse is what's bolded below:
> HTTPError: 400 Client Error: **home_page: Invalid URI** for url: http://pypi.org/legacy/
You've correctly found where the `home_page: <message>` bit comes from. The "Invalid URI" message comes from the form validation here: https://github.com/pypa/warehouse/blob/2a99b6f8b0ffa66a13d52b493c756cecc8307be1/warehouse/forms.py#L34 | 2018-01-11T17:54:28Z | [] | [] |
pypi/warehouse | 2,813 | pypi__warehouse-2813 | [
"1419"
] | 91d7820a2e3cf51b1649110d805188434ddb0347 | diff --git a/warehouse/packaging/models.py b/warehouse/packaging/models.py
--- a/warehouse/packaging/models.py
+++ b/warehouse/packaging/models.py
@@ -338,7 +338,7 @@ def urls(self):
_urls["Homepage"] = self.home_page
for urlspec in self.project_urls:
- name, url = urlspec.split(",", 1)
+ name, url = [x.strip() for x in urlspec.split(",", 1)]
_urls[name] = url
if self.download_url and "Download" not in _urls:
| diff --git a/tests/unit/packaging/test_models.py b/tests/unit/packaging/test_models.py
--- a/tests/unit/packaging/test_models.py
+++ b/tests/unit/packaging/test_models.py
@@ -159,6 +159,14 @@ def test_has_meta_false(self, db_session):
("Source Code", "https://example.com/source-code/"),
]),
),
+ (
+ None,
+ None,
+ ["Source Code, https://example.com/source-code/"],
+ OrderedDict([
+ ("Source Code", "https://example.com/source-code/"),
+ ]),
+ ),
(
"https://example.com/home/",
"https://example.com/download/",
| [Request] Package documentation link(s)
I'm following up [this issue on ReadTheDocs about browsing docs offline with 3rd party apps](https://github.com/rtfd/readthedocs.org/issues/662) - it looks like no one asked anyone here for help, but the issue is still outstanding.
There's currently no way to map from here to documentation in a format other applications can use. Details of why this would be useful are in the above ticket, but essentially there are examples of documentation names being different to the package names.
`pypi-legacy` accepts three URLs from a user, `Home page`, `Download URL` and `Bugtrack url`. `warehouse` only takes `url`, meant for the homepage.
Is there room for optional documentation URLs (plural as docs may be in multiple places and a user can choose which they prefer)?
[Also on pypi-legacy here](https://github.com/pypa/pypi-legacy/issues/501), as I'm not sure if this would be applied to `pypi-legacy` or kept for `warehouse`.
| Well warehouse takes Home page and download URL both currently. Both Warehouse and legacy also support arbitrary "Project URLs" which are essentially Name: URL pairs like: `"Documentation", "https://docs.pypi.org/"`, however setuptools doesn't currently expose a way to add those.
I may be wrong, but I think leaving it to a custom Project URL means developers won't include a documentation URL - there's no habit formed for including docs in Legacy, and there's no field there to serve as a reminder or indication that this could now be done, so few will know to, and even if they do it's still very easy to forget.
Also, @ewdurbin is happy to do this on Legacy, but if you decide a Product URL covers this then I don't think that can be done with Legacy.
What do you think?
I've proposed setuptools support for Project-URL metadata at https://github.com/pypa/setuptools/pull/1205 though my uploads to testpypi don't put the links anywhere obvious and the PKG-INFO link seems to present a munged metadata set with the Project-URL list stripped out (if you download the files however you can see it's present in both mudpy-0.0.1.dev231.dist-info/METADATA and mudpy-0.0.1.dev231/PKG-INFO files therein):
https://test.pypi.org/project/mudpy/
https://testpypi.python.org/pypi/mudpy/
The pkginfo library is able to find it in distributions downloaded from testpypi:
>>> pkginfo.SDist('mudpy-0.0.1.dev231.tar.gz').project_urls
[u'Bug Tracker, http://mudpy.org/contact/ Documentation, http://mudpy.org/documentation/ Source Code, http://mudpy.org/gitweb/mudpy.git']
The format of the list also seems to match the core metadata spec which in turn matches the design from PEP 345, so I'm not sure if I'm missing something or whether there's a bug in warehouse/cheeseshop.
There might be missing support for it, though I thought I added it.
Ahh, pouring back over the specs, I believe I've misinterpreted the described format. Multi-use means multiple lines beginning with "Project-URL:" rather than one with multiple indented Title,URL pairs on distinct lines. I'll rejigger the PR and do another test soon.
The respun Project-URL support for setuptools is now up for review as https://github.com/pypa/setuptools/pull/1210 and it's working for https://testpypi.python.org/pypi/mudpy/0.0.1.dev232/ but https://test.pypi.org/project/mudpy/0.0.1.dev232/ doesn't seem to provide the same links... leading me to suspect that implementation in Warehouse is not yet completed?
FWIW. I've the same problem with PyObjC: The wheels contain "Project-URL" metadata, but that doesn't show up on warehouse while pkginfo can extract the information. | 2018-01-18T20:36:41Z | [] | [] |
pypi/warehouse | 2,821 | pypi__warehouse-2821 | [
"2805"
] | 14a8c15524098fb9c23e1da7397dc0711b16634f | diff --git a/warehouse/admin/views/blacklist.py b/warehouse/admin/views/blacklist.py
--- a/warehouse/admin/views/blacklist.py
+++ b/warehouse/admin/views/blacklist.py
@@ -20,12 +20,12 @@
from sqlalchemy.orm.exc import NoResultFound
from warehouse.accounts.models import User
-from warehouse.admin.utils import remove_project
from warehouse.packaging.models import (
Project, Release, File, Role, BlacklistedProject
)
from warehouse.utils.http import is_safe_url
from warehouse.utils.paginate import paginate_url_factory
+from warehouse.utils.project import remove_project
@view_config(
diff --git a/warehouse/admin/views/projects.py b/warehouse/admin/views/projects.py
--- a/warehouse/admin/views/projects.py
+++ b/warehouse/admin/views/projects.py
@@ -22,9 +22,9 @@
from sqlalchemy import or_
from warehouse.accounts.models import User
-from warehouse.admin.utils import confirm_project, remove_project
from warehouse.packaging.models import Project, Release, Role, JournalEntry
from warehouse.utils.paginate import paginate_url_factory
+from warehouse.utils.project import confirm_project, remove_project
from warehouse.forklift.legacy import MAX_FILESIZE
ONE_MB = 1024 * 1024 # bytes
@@ -265,7 +265,7 @@ def set_upload_limit(project, request):
require_methods=False,
)
def delete_project(project, request):
- confirm_project(project, request)
+ confirm_project(project, request, fail_route="admin.project.detail")
remove_project(project, request)
return HTTPSeeOther(request.route_path('admin.project.list'))
diff --git a/warehouse/manage/views.py b/warehouse/manage/views.py
--- a/warehouse/manage/views.py
+++ b/warehouse/manage/views.py
@@ -22,6 +22,7 @@
from warehouse.accounts.models import User
from warehouse.manage.forms import CreateRoleForm, ChangeRoleForm
from warehouse.packaging.models import JournalEntry, Role
+from warehouse.utils.project import confirm_project, remove_project
@view_config(
@@ -46,7 +47,7 @@ def manage_projects(request):
@view_config(
route_name="manage.project.settings",
- renderer="manage/project.html",
+ renderer="manage/settings.html",
uses_session=True,
permission="manage",
effective_principals=Authenticated,
@@ -55,6 +56,30 @@ def manage_project_settings(project, request):
return {"project": project}
+@view_config(
+ route_name="manage.project.delete_project",
+ uses_session=True,
+ require_methods=["POST"],
+ permission="manage",
+)
+def delete_project(project, request):
+ confirm_project(project, request, fail_route="manage.project.settings")
+ remove_project(project, request)
+
+ return HTTPSeeOther(request.route_path('manage.projects'))
+
+
+@view_config(
+ route_name="manage.project.releases",
+ renderer="manage/releases.html",
+ uses_session=True,
+ permission="manage",
+ effective_principals=Authenticated,
+)
+def manage_project_releases(project, request):
+ return {"project": project}
+
+
@view_config(
route_name="manage.project.roles",
renderer="manage/roles.html",
@@ -208,7 +233,7 @@ def change_project_role(project, request, _form_class=ChangeRoleForm):
request.session.flash("Could not find role", queue="error")
return HTTPSeeOther(
- request.route_path('manage.project.roles', name=project.name)
+ request.route_path('manage.project.roles', project_name=project.name)
)
@@ -253,5 +278,5 @@ def delete_project_role(project, request):
request.session.flash("Successfully removed role", queue="success")
return HTTPSeeOther(
- request.route_path('manage.project.roles', name=project.name)
+ request.route_path('manage.project.roles', project_name=project.name)
)
diff --git a/warehouse/routes.py b/warehouse/routes.py
--- a/warehouse/routes.py
+++ b/warehouse/routes.py
@@ -110,30 +110,44 @@ def includeme(config):
config.add_route("manage.projects", "/manage/projects/", domain=warehouse)
config.add_route(
"manage.project.settings",
- "/manage/project/{name}/settings/",
+ "/manage/project/{project_name}/settings/",
factory="warehouse.packaging.models:ProjectFactory",
- traverse="/{name}",
+ traverse="/{project_name}",
+ domain=warehouse,
+ )
+ config.add_route(
+ "manage.project.delete_project",
+ "/manage/project/{project_name}/delete_project/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{project_name}",
+ domain=warehouse,
+ )
+ config.add_route(
+ "manage.project.releases",
+ "/manage/project/{project_name}/releases/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{project_name}",
domain=warehouse,
)
config.add_route(
"manage.project.roles",
- "/manage/project/{name}/collaboration/",
+ "/manage/project/{project_name}/collaboration/",
factory="warehouse.packaging.models:ProjectFactory",
- traverse="/{name}",
+ traverse="/{project_name}",
domain=warehouse,
)
config.add_route(
"manage.project.change_role",
- "/manage/project/{name}/collaboration/change/",
+ "/manage/project/{project_name}/collaboration/change/",
factory="warehouse.packaging.models:ProjectFactory",
- traverse="/{name}",
+ traverse="/{project_name}",
domain=warehouse,
)
config.add_route(
"manage.project.delete_role",
- "/manage/project/{name}/collaboration/delete/",
+ "/manage/project/{project_name}/collaboration/delete/",
factory="warehouse.packaging.models:ProjectFactory",
- traverse="/{name}",
+ traverse="/{project_name}",
domain=warehouse,
)
diff --git a/warehouse/admin/utils.py b/warehouse/utils/project.py
similarity index 90%
rename from warehouse/admin/utils.py
rename to warehouse/utils/project.py
--- a/warehouse/admin/utils.py
+++ b/warehouse/utils/project.py
@@ -18,7 +18,7 @@
)
-def confirm_project(project, request):
+def confirm_project(project, request, fail_route):
confirm = request.POST.get("confirm")
project_name = project.normalized_name
if not confirm:
@@ -27,10 +27,7 @@ def confirm_project(project, request):
queue="error",
)
raise HTTPSeeOther(
- request.route_path(
- 'admin.project.detail',
- project_name=project_name
- )
+ request.route_path(fail_route, project_name=project_name)
)
if canonicalize_name(confirm) != project.normalized_name:
request.session.flash(
@@ -38,10 +35,7 @@ def confirm_project(project, request):
queue="error",
)
raise HTTPSeeOther(
- request.route_path(
- 'admin.project.detail',
- project_name=project_name
- )
+ request.route_path(fail_route, project_name=project_name)
)
| diff --git a/tests/unit/manage/test_views.py b/tests/unit/manage/test_views.py
--- a/tests/unit/manage/test_views.py
+++ b/tests/unit/manage/test_views.py
@@ -13,13 +13,14 @@
import uuid
import pretend
+import pytest
from pyramid.httpexceptions import HTTPSeeOther
from webob.multidict import MultiDict
from warehouse.manage import views
from warehouse.accounts.interfaces import IUserService
-from warehouse.packaging.models import JournalEntry, Role
+from warehouse.packaging.models import JournalEntry, Project, Role
from ...common.db.packaging import ProjectFactory, RoleFactory, UserFactory
@@ -50,6 +51,84 @@ def test_manage_project_settings(self):
"project": project,
}
+ def test_delete_project_no_confirm(self):
+ project = pretend.stub(normalized_name='foo')
+ request = pretend.stub(
+ POST={},
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ ),
+ route_path=lambda *a, **kw: "/foo/bar/",
+ )
+
+ with pytest.raises(HTTPSeeOther) as exc:
+ views.delete_project(project, request)
+ assert exc.value.status_code == 303
+ assert exc.value.headers["Location"] == "/foo/bar/"
+
+ assert request.session.flash.calls == [
+ pretend.call("Must confirm the request.", queue="error"),
+ ]
+
+ def test_delete_project_wrong_confirm(self):
+ project = pretend.stub(normalized_name='foo')
+ request = pretend.stub(
+ POST={"confirm": "bar"},
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ ),
+ route_path=lambda *a, **kw: "/foo/bar/",
+ )
+
+ with pytest.raises(HTTPSeeOther) as exc:
+ views.delete_project(project, request)
+ assert exc.value.status_code == 303
+ assert exc.value.headers["Location"] == "/foo/bar/"
+
+ assert request.session.flash.calls == [
+ pretend.call("'bar' is not the same as 'foo'", queue="error"),
+ ]
+
+ def test_delete_project(self, db_request):
+ project = ProjectFactory.create(name="foo")
+
+ db_request.route_path = pretend.call_recorder(
+ lambda *a, **kw: "/the-redirect"
+ )
+ db_request.session = pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ )
+ db_request.POST["confirm"] = project.normalized_name
+ db_request.user = UserFactory.create()
+ db_request.remote_addr = "192.168.1.1"
+
+ result = views.delete_project(project, db_request)
+
+ assert db_request.session.flash.calls == [
+ pretend.call(
+ "Successfully deleted the project 'foo'.",
+ queue="success"
+ ),
+ ]
+ assert db_request.route_path.calls == [
+ pretend.call('manage.projects'),
+ ]
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/the-redirect"
+ assert not (db_request.db.query(Project)
+ .filter(Project.name == "foo").count())
+
+
+class TestManageProjectReleases:
+
+ def test_manage_project_releases(self):
+ request = pretend.stub()
+ project = pretend.stub()
+
+ assert views.manage_project_releases(project, request) == {
+ "project": project,
+ }
+
class TestManageProjectRoles:
@@ -76,7 +155,6 @@ def test_get_manage_project_roles(self, db_request):
pretend.call(db_request.POST, user_service=user_service),
]
assert result == {
-
"project": project,
"roles_by_user": {user.username: [role]},
"form": form_obj,
@@ -252,7 +330,7 @@ def test_change_role(self, db_request):
assert role.role_name == new_role_name
assert db_request.route_path.calls == [
- pretend.call('manage.project.roles', name=project.name),
+ pretend.call('manage.project.roles', project_name=project.name),
]
assert db_request.session.flash.calls == [
pretend.call("Successfully changed role", queue="success"),
@@ -282,7 +360,7 @@ def test_change_role_invalid_role_name(self, pyramid_request):
result = views.change_project_role(project, pyramid_request)
assert pyramid_request.route_path.calls == [
- pretend.call('manage.project.roles', name=project.name),
+ pretend.call('manage.project.roles', project_name=project.name),
]
assert isinstance(result, HTTPSeeOther)
assert result.headers["Location"] == "/the-redirect"
@@ -317,7 +395,7 @@ def test_change_role_when_multiple(self, db_request):
assert db_request.db.query(Role).all() == [maintainer_role]
assert db_request.route_path.calls == [
- pretend.call('manage.project.roles', name=project.name),
+ pretend.call('manage.project.roles', project_name=project.name),
]
assert db_request.session.flash.calls == [
pretend.call("Successfully changed role", queue="success"),
@@ -441,7 +519,7 @@ def test_delete_role(self, db_request):
result = views.delete_project_role(project, db_request)
assert db_request.route_path.calls == [
- pretend.call('manage.project.roles', name=project.name),
+ pretend.call('manage.project.roles', project_name=project.name),
]
assert db_request.db.query(Role).all() == []
assert db_request.session.flash.calls == [
diff --git a/tests/unit/test_routes.py b/tests/unit/test_routes.py
--- a/tests/unit/test_routes.py
+++ b/tests/unit/test_routes.py
@@ -144,30 +144,44 @@ def add_policy(name, filename):
),
pretend.call(
"manage.project.settings",
- "/manage/project/{name}/settings/",
+ "/manage/project/{project_name}/settings/",
factory="warehouse.packaging.models:ProjectFactory",
- traverse="/{name}",
+ traverse="/{project_name}",
+ domain=warehouse,
+ ),
+ pretend.call(
+ "manage.project.delete_project",
+ "/manage/project/{project_name}/delete_project/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{project_name}",
+ domain=warehouse,
+ ),
+ pretend.call(
+ "manage.project.releases",
+ "/manage/project/{project_name}/releases/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{project_name}",
domain=warehouse,
),
pretend.call(
"manage.project.roles",
- "/manage/project/{name}/collaboration/",
+ "/manage/project/{project_name}/collaboration/",
factory="warehouse.packaging.models:ProjectFactory",
- traverse="/{name}",
+ traverse="/{project_name}",
domain=warehouse,
),
pretend.call(
"manage.project.change_role",
- "/manage/project/{name}/collaboration/change/",
+ "/manage/project/{project_name}/collaboration/change/",
factory="warehouse.packaging.models:ProjectFactory",
- traverse="/{name}",
+ traverse="/{project_name}",
domain=warehouse,
),
pretend.call(
"manage.project.delete_role",
- "/manage/project/{name}/collaboration/delete/",
+ "/manage/project/{project_name}/collaboration/delete/",
factory="warehouse.packaging.models:ProjectFactory",
- traverse="/{name}",
+ traverse="/{project_name}",
domain=warehouse,
),
pretend.call(
diff --git a/tests/unit/admin/test_utils.py b/tests/unit/utils/test_project.py
similarity index 91%
rename from tests/unit/admin/test_utils.py
rename to tests/unit/utils/test_project.py
--- a/tests/unit/admin/test_utils.py
+++ b/tests/unit/utils/test_project.py
@@ -14,10 +14,10 @@
from pretend import call, call_recorder, stub
from pyramid.httpexceptions import HTTPSeeOther
-from warehouse.admin.utils import confirm_project, remove_project
from warehouse.packaging.models import (
Project, Release, Dependency, File, Role, JournalEntry
)
+from warehouse.utils.project import confirm_project, remove_project
from ...common.db.accounts import UserFactory
from ...common.db.packaging import (
@@ -34,7 +34,7 @@ def test_confirm():
session=stub(flash=call_recorder(lambda *a, **kw: stub())),
)
- confirm_project(project, request)
+ confirm_project(project, request, fail_route='fail_route')
assert request.route_path.calls == []
assert request.session.flash.calls == []
@@ -49,11 +49,11 @@ def test_confirm_no_input():
)
with pytest.raises(HTTPSeeOther) as err:
- confirm_project(project, request)
+ confirm_project(project, request, fail_route='fail_route')
assert err.value == '/the-redirect'
assert request.route_path.calls == [
- call('admin.project.detail', project_name='foobar')
+ call('fail_route', project_name='foobar')
]
assert request.session.flash.calls == [
call('Must confirm the request.', queue='error')
@@ -69,11 +69,11 @@ def test_confirm_incorrect_input():
)
with pytest.raises(HTTPSeeOther) as err:
- confirm_project(project, request)
+ confirm_project(project, request, fail_route='fail_route')
assert err.value == '/the-redirect'
assert request.route_path.calls == [
- call('admin.project.detail', project_name='foobar')
+ call('fail_route', project_name='foobar')
]
assert request.session.flash.calls == [
call("'bizbaz' is not the same as 'foobar'", queue='error')
| Enable deleting a project
A sub ticket of #424
We need to enable deleting a project via the manager admin.
I have created the UI for this in `warehouse/templates/manage/project.html`
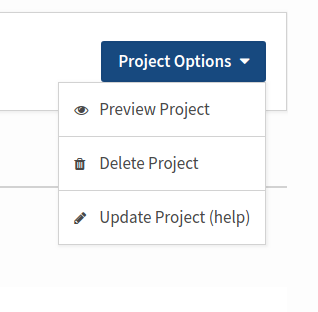
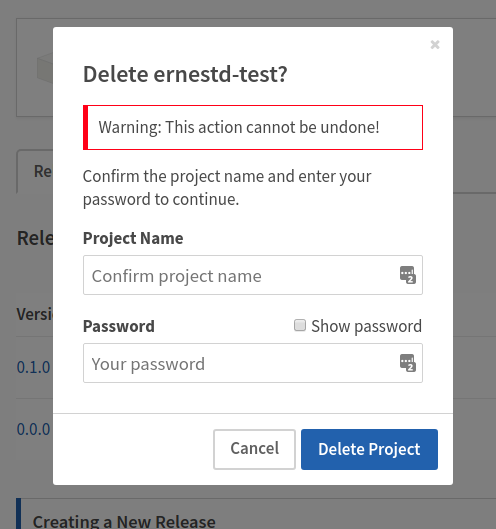
The code for this is currently commented out.
We need to:
- Uncomment the relevant html sections
- Make the delete form (in modal) work, including the 'show password' functionality
- Add a redirect (to the projects page?), that includes a confirmation message to tell the user their project has been deleted successfully.
| 2018-01-22T21:16:54Z | [] | [] |
|
pypi/warehouse | 2,823 | pypi__warehouse-2823 | [
"2810"
] | ca2bf8bfcbe60914d6f1ed7925efe52931135515 | diff --git a/warehouse/packaging/views.py b/warehouse/packaging/views.py
--- a/warehouse/packaging/views.py
+++ b/warehouse/packaging/views.py
@@ -126,3 +126,13 @@ def release_detail(release, request):
"maintainers": maintainers,
"license": license,
}
+
+
+@view_config(
+ route_name="includes.edit-project-button",
+ renderer="includes/edit-project-button.html",
+ uses_session=True,
+ permission="manage",
+)
+def edit_project_button(project, request):
+ return {'project': project}
diff --git a/warehouse/routes.py b/warehouse/routes.py
--- a/warehouse/routes.py
+++ b/warehouse/routes.py
@@ -68,6 +68,13 @@ def includeme(config):
traverse="/{username}",
domain=warehouse,
)
+ config.add_route(
+ "includes.edit-project-button",
+ "/_includes/edit-project-button/{project_name}",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{project_name}",
+ domain=warehouse,
+ )
# Search Routes
config.add_route("search", "/search/", domain=warehouse)
| diff --git a/tests/unit/packaging/test_views.py b/tests/unit/packaging/test_views.py
--- a/tests/unit/packaging/test_views.py
+++ b/tests/unit/packaging/test_views.py
@@ -231,3 +231,12 @@ def test_multiple_licenses_from_classifiers(self, db_request):
result = views.release_detail(release, db_request)
assert result["license"] == "BSD License, MIT License"
+
+
+class TestEditProjectButton:
+
+ def test_edit_project_button_returns_project(self):
+ project = pretend.stub()
+ assert views.edit_project_button(project, pretend.stub()) == {
+ 'project': project
+ }
diff --git a/tests/unit/test_routes.py b/tests/unit/test_routes.py
--- a/tests/unit/test_routes.py
+++ b/tests/unit/test_routes.py
@@ -100,6 +100,13 @@ def add_policy(name, filename):
traverse="/{username}",
domain=warehouse,
),
+ pretend.call(
+ "includes.edit-project-button",
+ "/_includes/edit-project-button/{project_name}",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{project_name}",
+ domain=warehouse,
+ ),
pretend.call("search", "/search/", domain=warehouse),
pretend.call(
"accounts.profile",
| Link to 'edit project' when logged in user is owner
I have added a button in the UI to link *back* the the manage project page, when the user is viewing their project.
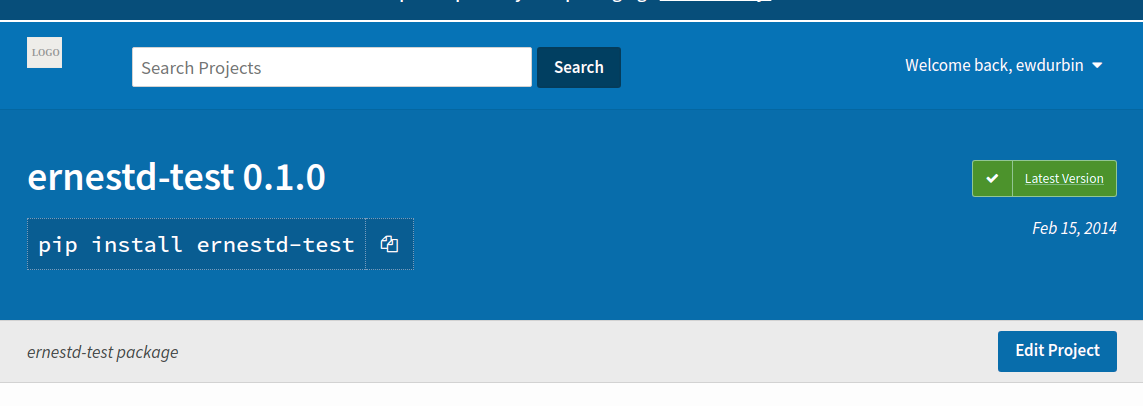
This is currently commented out.
| A sub ticket of #424
This will need it's own client-side-include route. | 2018-01-22T22:36:09Z | [] | [] |
pypi/warehouse | 2,833 | pypi__warehouse-2833 | [
"2829"
] | a8920b63a4e006074ad9e274298e226495a6d57b | diff --git a/warehouse/accounts/views.py b/warehouse/accounts/views.py
--- a/warehouse/accounts/views.py
+++ b/warehouse/accounts/views.py
@@ -371,3 +371,12 @@ def _login_user(request, userid):
)
def profile_callout(user, request):
return {"user": user}
+
+
+@view_config(
+ route_name="includes.edit-profile-button",
+ renderer="includes/accounts/edit-profile-button.html",
+ uses_session=True,
+)
+def edit_profile_button(request):
+ return {}
diff --git a/warehouse/manage/forms.py b/warehouse/manage/forms.py
--- a/warehouse/manage/forms.py
+++ b/warehouse/manage/forms.py
@@ -55,3 +55,10 @@ def __init__(self, *args, user_service, **kwargs):
class ChangeRoleForm(RoleNameMixin, forms.Form):
pass
+
+
+class SaveProfileForm(forms.Form):
+
+ __params__ = ['name']
+
+ name = wtforms.StringField()
diff --git a/warehouse/manage/views.py b/warehouse/manage/views.py
--- a/warehouse/manage/views.py
+++ b/warehouse/manage/views.py
@@ -15,24 +15,53 @@
from pyramid.httpexceptions import HTTPSeeOther
from pyramid.security import Authenticated
-from pyramid.view import view_config
+from pyramid.view import view_config, view_defaults
from sqlalchemy.orm.exc import NoResultFound
from warehouse.accounts.interfaces import IUserService
from warehouse.accounts.models import User
-from warehouse.manage.forms import CreateRoleForm, ChangeRoleForm
+from warehouse.manage.forms import (
+ CreateRoleForm, ChangeRoleForm, SaveProfileForm
+)
from warehouse.packaging.models import JournalEntry, Role
from warehouse.utils.project import confirm_project, remove_project
-@view_config(
+@view_defaults(
route_name="manage.profile",
renderer="manage/profile.html",
uses_session=True,
+ require_csrf=True,
+ require_methods=False,
effective_principals=Authenticated,
)
-def manage_profile(request):
- return {}
+class ManageProfileViews:
+ def __init__(self, request):
+ self.request = request
+ self.user_service = request.find_service(IUserService, context=None)
+
+ @view_config(request_method="GET")
+ def manage_profile(self):
+ return {
+ 'save_profile_form': SaveProfileForm(name=self.request.user.name),
+ }
+
+ @view_config(
+ request_method="POST",
+ request_param=SaveProfileForm.__params__,
+ )
+ def save_profile(self):
+ form = SaveProfileForm(self.request.POST)
+
+ if form.validate():
+ self.user_service.update_user(self.request.user.id, **form.data)
+ self.request.session.flash(
+ 'Public profile updated.', queue='success'
+ )
+
+ return {
+ 'save_profile_form': form,
+ }
@view_config(
diff --git a/warehouse/routes.py b/warehouse/routes.py
--- a/warehouse/routes.py
+++ b/warehouse/routes.py
@@ -75,6 +75,11 @@ def includeme(config):
traverse="/{project_name}",
domain=warehouse,
)
+ config.add_route(
+ "includes.edit-profile-button",
+ "/_includes/edit-profile-button/",
+ domain=warehouse,
+ )
# Search Routes
config.add_route("search", "/search/", domain=warehouse)
| diff --git a/tests/unit/accounts/test_views.py b/tests/unit/accounts/test_views.py
--- a/tests/unit/accounts/test_views.py
+++ b/tests/unit/accounts/test_views.py
@@ -566,3 +566,11 @@ def test_profile_callout_returns_user(self):
request = pretend.stub()
assert views.profile_callout(user, request) == {"user": user}
+
+
+class TestEditProfileButton:
+
+ def test_edit_profile_button(self):
+ request = pretend.stub()
+
+ assert views.edit_profile_button(request) == {}
diff --git a/tests/unit/manage/test_views.py b/tests/unit/manage/test_views.py
--- a/tests/unit/manage/test_views.py
+++ b/tests/unit/manage/test_views.py
@@ -27,10 +27,85 @@
class TestManageProfile:
- def test_manage_profile(self):
- request = pretend.stub()
+ def test_manage_profile(self, monkeypatch):
+ user_service = pretend.stub()
+ name = pretend.stub()
+ request = pretend.stub(
+ find_service=lambda *a, **kw: user_service,
+ user=pretend.stub(name=name),
+ )
+ save_profile_obj = pretend.stub()
+ save_profile_cls = pretend.call_recorder(lambda **kw: save_profile_obj)
+ monkeypatch.setattr(views, 'SaveProfileForm', save_profile_cls)
+ view_class = views.ManageProfileViews(request)
+
+ assert view_class.manage_profile() == {
+ 'save_profile_form': save_profile_obj,
+ }
+ assert view_class.request == request
+ assert view_class.user_service == user_service
+ assert save_profile_cls.calls == [
+ pretend.call(name=name),
+ ]
+
+ def test_save_profile(self, monkeypatch):
+ update_user = pretend.call_recorder(lambda *a, **kw: None)
+ request = pretend.stub(
+ POST={'name': 'new name'},
+ user=pretend.stub(id=pretend.stub()),
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ ),
+ find_service=pretend.call_recorder(
+ lambda iface, context: pretend.stub(update_user=update_user)
+ ),
+ )
+ save_profile_obj = pretend.stub(
+ validate=lambda: True,
+ data=request.POST,
+ )
+ save_profile_cls = pretend.call_recorder(lambda d: save_profile_obj)
+ monkeypatch.setattr(views, 'SaveProfileForm', save_profile_cls)
+ view_class = views.ManageProfileViews(request)
- assert views.manage_profile(request) == {}
+ assert view_class.save_profile() == {
+ 'save_profile_form': save_profile_obj,
+ }
+ assert request.session.flash.calls == [
+ pretend.call('Public profile updated.', queue='success'),
+ ]
+ assert update_user.calls == [
+ pretend.call(request.user.id, **request.POST)
+ ]
+ assert save_profile_cls.calls == [
+ pretend.call(request.POST),
+ ]
+
+ def test_save_profile_validation_fails(self, monkeypatch):
+ update_user = pretend.call_recorder(lambda *a, **kw: None)
+ request = pretend.stub(
+ POST={'name': 'new name'},
+ user=pretend.stub(id=pretend.stub()),
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ ),
+ find_service=pretend.call_recorder(
+ lambda iface, context: pretend.stub(update_user=update_user)
+ ),
+ )
+ save_profile_obj = pretend.stub(validate=lambda: False)
+ save_profile_cls = pretend.call_recorder(lambda d: save_profile_obj)
+ monkeypatch.setattr(views, 'SaveProfileForm', save_profile_cls)
+ view_class = views.ManageProfileViews(request)
+
+ assert view_class.save_profile() == {
+ 'save_profile_form': save_profile_obj,
+ }
+ assert request.session.flash.calls == []
+ assert update_user.calls == []
+ assert save_profile_cls.calls == [
+ pretend.call(request.POST),
+ ]
class TestManageProjects:
diff --git a/tests/unit/test_routes.py b/tests/unit/test_routes.py
--- a/tests/unit/test_routes.py
+++ b/tests/unit/test_routes.py
@@ -107,6 +107,11 @@ def add_policy(name, filename):
traverse="/{project_name}",
domain=warehouse,
),
+ pretend.call(
+ "includes.edit-profile-button",
+ "/_includes/edit-profile-button/",
+ domain=warehouse,
+ ),
pretend.call("search", "/search/", domain=warehouse),
pretend.call(
"accounts.profile",
| Manage Account: Change public information
From #423: From the 'manage account' page, a logged-in user should be able to change the following information displayed on their "public" profile:
* Gravatar image (display the image and provide a link to gravatar to change it)
* Name
| 2018-01-23T22:16:05Z | [] | [] |
|
pypi/warehouse | 2,846 | pypi__warehouse-2846 | [
"2843"
] | d61cf86de5f37420575e5e411d193a0fb9c9e7f9 | diff --git a/warehouse/views.py b/warehouse/views.py
--- a/warehouse/views.py
+++ b/warehouse/views.py
@@ -125,6 +125,14 @@ def forbidden(exc, request, redirect_to="accounts.login"):
return httpexception_view(exc, request)
+@forbidden_view_config(path_info=r"^/_includes/")
+@exception_view_config(PredicateMismatch, path_info=r"^/_includes/")
+def forbidden_include(exc, request):
+ # If the forbidden error is for a client-side-include, just return an empty
+ # response instead of redirecting
+ return Response()
+
+
@view_config(
route_name="robots.txt",
renderer="robots.txt",
| diff --git a/tests/unit/test_views.py b/tests/unit/test_views.py
--- a/tests/unit/test_views.py
+++ b/tests/unit/test_views.py
@@ -25,7 +25,7 @@
from warehouse.views import (
SEARCH_BOOSTS, SEARCH_FIELDS, current_user_indicator, forbidden, health,
httpexception_view, index, robotstxt, opensearchxml, search, force_status,
- flash_messages
+ flash_messages, forbidden_include
)
from ..common.db.accounts import UserFactory
@@ -147,6 +147,19 @@ def test_logged_out_redirects_login(self):
"/accounts/login/?next=/foo/bar/%3Fb%3Ds"
+class TestForbiddenIncludeView:
+
+ def test_forbidden_include(self):
+ exc = pretend.stub()
+ request = pretend.stub()
+
+ resp = forbidden_include(exc, request)
+
+ assert resp.status_code == 200
+ assert resp.content_type == 'text/html'
+ assert resp.content_length == 0
+
+
def test_robotstxt(pyramid_request):
assert robotstxt(pyramid_request) == {}
assert pyramid_request.response.content_type == "text/plain"
| duplicate footer appears in package page only when not logged in
When viewing a package page (appears to apply to any package), the (blue) footer ("Get Help", "About PyPI", "Contributing to PyPI") occurs twice: once below a login prompt and a search text box that appear below the package's title (with the package details below these and the footer), and once at the bottom of the page (the latter as expected).
This behavior occurs only when logged out. In contrast, when logged in, the layout seems as intended: a search text box at the top, and the package title and details contiguously below that search box, with a single footer at the bottom.
| <img width="565" alt="screen shot 2018-01-26 at 7 05 03 pm" src="https://user-images.githubusercontent.com/3275593/35442066-dc315d82-02cb-11e8-8eeb-d879a8181cab.png">
Indeed, this is how it looks, with the bottom footer appearing after the section of details that starts with "Navigation". Thanks for adding a picture.
Going to guess an HTML include got messed up, i think @di added one here recently?
Yeah, I know what's causing this. On it. | 2018-01-26T15:37:00Z | [] | [] |
pypi/warehouse | 2,879 | pypi__warehouse-2879 | [
"424",
"2808"
] | 595a318bbe59bd760f89e6f2421ecf0dda430d18 | diff --git a/warehouse/manage/views.py b/warehouse/manage/views.py
--- a/warehouse/manage/views.py
+++ b/warehouse/manage/views.py
@@ -23,7 +23,7 @@
from warehouse.manage.forms import (
CreateRoleForm, ChangeRoleForm, SaveProfileForm
)
-from warehouse.packaging.models import JournalEntry, Role
+from warehouse.packaging.models import JournalEntry, Role, File
from warehouse.utils.project import confirm_project, remove_project
@@ -109,6 +109,151 @@ def manage_project_releases(project, request):
return {"project": project}
+@view_defaults(
+ route_name="manage.project.release",
+ renderer="manage/release.html",
+ uses_session=True,
+ require_csrf=True,
+ require_methods=False,
+ permission="manage",
+ effective_principals=Authenticated,
+)
+class ManageProjectRelease:
+ def __init__(self, release, request):
+ self.release = release
+ self.request = request
+
+ @view_config(request_method="GET")
+ def manage_project_release(self):
+ return {
+ "project": self.release.project,
+ "release": self.release,
+ "files": self.release.files.all(),
+ }
+
+ @view_config(
+ request_method="POST",
+ request_param=["confirm_version"]
+ )
+ def delete_project_release(self):
+ version = self.request.POST.get('confirm_version')
+ if not version:
+ self.request.session.flash(
+ "Must confirm the request.", queue='error'
+ )
+ return HTTPSeeOther(
+ self.request.route_path(
+ 'manage.project.release',
+ project_name=self.release.project.name,
+ version=self.release.version,
+ )
+ )
+
+ if version != self.release.version:
+ self.request.session.flash(
+ "Could not delete release - " +
+ f"{version!r} is not the same as {self.release.version!r}",
+ queue="error",
+ )
+ return HTTPSeeOther(
+ self.request.route_path(
+ 'manage.project.release',
+ project_name=self.release.project.name,
+ version=self.release.version,
+ )
+ )
+
+ self.request.db.add(
+ JournalEntry(
+ name=self.release.project.name,
+ action="remove",
+ version=self.release.version,
+ submitted_by=self.request.user,
+ submitted_from=self.request.remote_addr,
+ ),
+ )
+
+ self.request.db.delete(self.release)
+
+ self.request.session.flash(
+ f"Successfully deleted release {self.release.version!r}.",
+ queue="success",
+ )
+
+ return HTTPSeeOther(
+ self.request.route_path(
+ 'manage.project.releases',
+ project_name=self.release.project.name,
+ )
+ )
+
+ @view_config(
+ request_method="POST",
+ request_param=["confirm_filename", "file_id"]
+ )
+ def delete_project_release_file(self):
+ filename = self.request.POST.get('confirm_filename')
+ if not filename:
+ self.request.session.flash(
+ "Must confirm the request.", queue='error'
+ )
+ return HTTPSeeOther(
+ self.request.route_path(
+ 'manage.project.release',
+ project_name=self.release.project.name,
+ version=self.release.version,
+ )
+ )
+
+ release_file = (
+ self.request.db.query(File)
+ .filter(
+ File.name == self.release.project.name,
+ File.id == self.request.POST.get('file_id'),
+ )
+ .one()
+ )
+
+ if filename != release_file.filename:
+ self.request.session.flash(
+ "Could not delete file - " +
+ f"{filename!r} is not the same as {release_file.filename!r}",
+ queue="error",
+ )
+ return HTTPSeeOther(
+ self.request.route_path(
+ 'manage.project.release',
+ project_name=self.release.project.name,
+ version=self.release.version,
+ )
+ )
+
+ self.request.db.add(
+ JournalEntry(
+ name=self.release.project.name,
+ action=f"remove file {release_file.filename}",
+ version=self.release.version,
+ submitted_by=self.request.user,
+ submitted_from=self.request.remote_addr,
+ ),
+ )
+
+ self.request.db.delete(release_file)
+
+ self.request.session.flash(
+ f"Successfully deleted file {release_file.filename!r}.",
+ queue="success",
+ )
+
+ return HTTPSeeOther(
+ self.request.route_path(
+ 'manage.project.release',
+ project_name=self.release.project.name,
+ version=self.release.version,
+ )
+ )
+
+
@view_config(
route_name="manage.project.roles",
renderer="manage/roles.html",
diff --git a/warehouse/packaging/models.py b/warehouse/packaging/models.py
--- a/warehouse/packaging/models.py
+++ b/warehouse/packaging/models.py
@@ -342,6 +342,25 @@ def __table_args__(cls): # noqa
viewonly=True,
)
+ def __acl__(self):
+ session = orm.object_session(self)
+ acls = [
+ (Allow, "group:admins", "admin"),
+ ]
+
+ # Get all of the users for this project.
+ query = session.query(Role).filter(Role.project == self)
+ query = query.options(orm.lazyload("project"))
+ query = query.options(orm.joinedload("user").lazyload("emails"))
+ for role in sorted(
+ query.all(),
+ key=lambda x: ["Owner", "Maintainer"].index(x.role_name)):
+ if role.role_name == "Owner":
+ acls.append((Allow, str(role.user.id), ["manage", "upload"]))
+ else:
+ acls.append((Allow, str(role.user.id), ["upload"]))
+ return acls
+
@property
def urls(self):
_urls = OrderedDict()
diff --git a/warehouse/routes.py b/warehouse/routes.py
--- a/warehouse/routes.py
+++ b/warehouse/routes.py
@@ -134,6 +134,13 @@ def includeme(config):
traverse="/{project_name}",
domain=warehouse,
)
+ config.add_route(
+ "manage.project.release",
+ "/manage/project/{project_name}/release/{version}/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{project_name}/{version}",
+ domain=warehouse,
+ )
config.add_route(
"manage.project.roles",
"/manage/project/{project_name}/collaboration/",
| diff --git a/tests/unit/manage/test_views.py b/tests/unit/manage/test_views.py
--- a/tests/unit/manage/test_views.py
+++ b/tests/unit/manage/test_views.py
@@ -208,6 +208,297 @@ def test_manage_project_releases(self):
}
+class TestManageProjectRelease:
+
+ def test_manage_project_release(self):
+ files = pretend.stub()
+ project = pretend.stub()
+ release = pretend.stub(
+ project=project,
+ files=pretend.stub(all=lambda: files),
+ )
+ request = pretend.stub()
+ view = views.ManageProjectRelease(release, request)
+
+ assert view.manage_project_release() == {
+ 'project': project,
+ 'release': release,
+ 'files': files,
+ }
+
+ def test_delete_project_release(self, monkeypatch):
+ release = pretend.stub(
+ version='1.2.3',
+ project=pretend.stub(name='foobar'),
+ )
+ request = pretend.stub(
+ POST={'confirm_version': release.version},
+ method="POST",
+ db=pretend.stub(
+ delete=pretend.call_recorder(lambda a: None),
+ add=pretend.call_recorder(lambda a: None),
+ ),
+ route_path=pretend.call_recorder(lambda *a, **kw: '/the-redirect'),
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None)
+ ),
+ user=pretend.stub(),
+ remote_addr=pretend.stub(),
+ )
+ journal_obj = pretend.stub()
+ journal_cls = pretend.call_recorder(lambda **kw: journal_obj)
+ monkeypatch.setattr(views, 'JournalEntry', journal_cls)
+
+ view = views.ManageProjectRelease(release, request)
+
+ result = view.delete_project_release()
+
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/the-redirect"
+
+ assert request.db.delete.calls == [pretend.call(release)]
+ assert request.db.add.calls == [pretend.call(journal_obj)]
+ assert journal_cls.calls == [
+ pretend.call(
+ name=release.project.name,
+ action="remove",
+ version=release.version,
+ submitted_by=request.user,
+ submitted_from=request.remote_addr,
+ ),
+ ]
+ assert request.session.flash.calls == [
+ pretend.call(
+ f"Successfully deleted release {release.version!r}.",
+ queue="success",
+ )
+ ]
+ assert request.route_path.calls == [
+ pretend.call(
+ 'manage.project.releases',
+ project_name=release.project.name,
+ )
+ ]
+
+ def test_delete_project_release_no_confirm(self):
+ release = pretend.stub(
+ version='1.2.3',
+ project=pretend.stub(name='foobar'),
+ )
+ request = pretend.stub(
+ POST={'confirm_version': ''},
+ method="POST",
+ db=pretend.stub(delete=pretend.call_recorder(lambda a: None)),
+ route_path=pretend.call_recorder(lambda *a, **kw: '/the-redirect'),
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None)
+ ),
+ )
+ view = views.ManageProjectRelease(release, request)
+
+ result = view.delete_project_release()
+
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/the-redirect"
+
+ assert request.db.delete.calls == []
+ assert request.session.flash.calls == [
+ pretend.call(
+ "Must confirm the request.", queue='error'
+ )
+ ]
+ assert request.route_path.calls == [
+ pretend.call(
+ 'manage.project.release',
+ project_name=release.project.name,
+ version=release.version,
+ )
+ ]
+
+ def test_delete_project_release_bad_confirm(self):
+ release = pretend.stub(
+ version='1.2.3',
+ project=pretend.stub(name='foobar'),
+ )
+ request = pretend.stub(
+ POST={'confirm_version': 'invalid'},
+ method="POST",
+ db=pretend.stub(delete=pretend.call_recorder(lambda a: None)),
+ route_path=pretend.call_recorder(lambda *a, **kw: '/the-redirect'),
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None)
+ ),
+ )
+ view = views.ManageProjectRelease(release, request)
+
+ result = view.delete_project_release()
+
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/the-redirect"
+
+ assert request.db.delete.calls == []
+ assert request.session.flash.calls == [
+ pretend.call(
+ "Could not delete release - " +
+ f"'invalid' is not the same as {release.version!r}",
+ queue="error",
+ )
+ ]
+ assert request.route_path.calls == [
+ pretend.call(
+ 'manage.project.release',
+ project_name=release.project.name,
+ version=release.version,
+ )
+ ]
+
+ def test_delete_project_release_file(self, monkeypatch):
+ release_file = pretend.stub(
+ filename='foo-bar.tar.gz',
+ id=str(uuid.uuid4()),
+ )
+ release = pretend.stub(
+ version='1.2.3',
+ project=pretend.stub(name='foobar'),
+ )
+ request = pretend.stub(
+ POST={
+ 'confirm_filename': release_file.filename,
+ 'file_id': release_file.id,
+ },
+ method="POST",
+ db=pretend.stub(
+ delete=pretend.call_recorder(lambda a: None),
+ add=pretend.call_recorder(lambda a: None),
+ query=lambda a: pretend.stub(
+ filter=lambda *a: pretend.stub(one=lambda: release_file),
+ ),
+ ),
+ route_path=pretend.call_recorder(lambda *a, **kw: '/the-redirect'),
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None)
+ ),
+ user=pretend.stub(),
+ remote_addr=pretend.stub(),
+ )
+ journal_obj = pretend.stub()
+ journal_cls = pretend.call_recorder(lambda **kw: journal_obj)
+ monkeypatch.setattr(views, 'JournalEntry', journal_cls)
+
+ view = views.ManageProjectRelease(release, request)
+
+ result = view.delete_project_release_file()
+
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/the-redirect"
+
+ assert request.session.flash.calls == [
+ pretend.call(
+ f"Successfully deleted file {release_file.filename!r}.",
+ queue="success",
+ )
+ ]
+ assert request.db.delete.calls == [pretend.call(release_file)]
+ assert request.db.add.calls == [pretend.call(journal_obj)]
+ assert journal_cls.calls == [
+ pretend.call(
+ name=release.project.name,
+ action=f"remove file {release_file.filename}",
+ version=release.version,
+ submitted_by=request.user,
+ submitted_from=request.remote_addr,
+ ),
+ ]
+ assert request.route_path.calls == [
+ pretend.call(
+ 'manage.project.release',
+ project_name=release.project.name,
+ version=release.version,
+ )
+ ]
+
+ def test_delete_project_release_file_no_confirm(self):
+ release = pretend.stub(
+ version='1.2.3',
+ project=pretend.stub(name='foobar'),
+ )
+ request = pretend.stub(
+ POST={'confirm_filename': ''},
+ method="POST",
+ db=pretend.stub(delete=pretend.call_recorder(lambda a: None)),
+ route_path=pretend.call_recorder(lambda *a, **kw: '/the-redirect'),
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None)
+ ),
+ )
+ view = views.ManageProjectRelease(release, request)
+
+ result = view.delete_project_release_file()
+
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/the-redirect"
+
+ assert request.db.delete.calls == []
+ assert request.session.flash.calls == [
+ pretend.call(
+ "Must confirm the request.", queue='error'
+ )
+ ]
+ assert request.route_path.calls == [
+ pretend.call(
+ 'manage.project.release',
+ project_name=release.project.name,
+ version=release.version,
+ )
+ ]
+
+ def test_delete_project_release_file_bad_confirm(self):
+ release_file = pretend.stub(
+ filename='foo-bar.tar.gz',
+ id=str(uuid.uuid4()),
+ )
+ release = pretend.stub(
+ version='1.2.3',
+ project=pretend.stub(name='foobar'),
+ )
+ request = pretend.stub(
+ POST={'confirm_filename': 'invalid'},
+ method="POST",
+ db=pretend.stub(
+ delete=pretend.call_recorder(lambda a: None),
+ query=lambda a: pretend.stub(
+ filter=lambda *a: pretend.stub(one=lambda: release_file),
+ ),
+ ),
+ route_path=pretend.call_recorder(lambda *a, **kw: '/the-redirect'),
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None)
+ ),
+ )
+ view = views.ManageProjectRelease(release, request)
+
+ result = view.delete_project_release_file()
+
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/the-redirect"
+
+ assert request.db.delete.calls == []
+ assert request.session.flash.calls == [
+ pretend.call(
+ "Could not delete file - " +
+ f"'invalid' is not the same as {release_file.filename!r}",
+ queue="error",
+ )
+ ]
+ assert request.route_path.calls == [
+ pretend.call(
+ 'manage.project.release',
+ project_name=release.project.name,
+ version=release.version,
+ )
+ ]
+
+
class TestManageProjectRoles:
def test_get_manage_project_roles(self, db_request):
diff --git a/tests/unit/packaging/test_models.py b/tests/unit/packaging/test_models.py
--- a/tests/unit/packaging/test_models.py
+++ b/tests/unit/packaging/test_models.py
@@ -254,6 +254,28 @@ def test_urls(self, db_session, home_page, download_url, project_urls,
# TODO: It'd be nice to test for the actual ordering here.
assert dict(release.urls) == dict(expected)
+ def test_acl(self, db_session):
+ project = DBProjectFactory.create()
+ owner1 = DBRoleFactory.create(project=project)
+ owner2 = DBRoleFactory.create(project=project)
+ maintainer1 = DBRoleFactory.create(
+ project=project,
+ role_name="Maintainer",
+ )
+ maintainer2 = DBRoleFactory.create(
+ project=project,
+ role_name="Maintainer",
+ )
+ release = DBReleaseFactory.create(project=project)
+
+ assert release.__acl__() == [
+ (Allow, "group:admins", "admin"),
+ (Allow, str(owner1.user.id), ["manage", "upload"]),
+ (Allow, str(owner2.user.id), ["manage", "upload"]),
+ (Allow, str(maintainer1.user.id), ["upload"]),
+ (Allow, str(maintainer2.user.id), ["upload"]),
+ ]
+
class TestFile:
diff --git a/tests/unit/test_routes.py b/tests/unit/test_routes.py
--- a/tests/unit/test_routes.py
+++ b/tests/unit/test_routes.py
@@ -168,6 +168,13 @@ def add_policy(name, filename):
traverse="/{project_name}",
domain=warehouse,
),
+ pretend.call(
+ "manage.project.release",
+ "/manage/project/{project_name}/release/{version}/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{project_name}/{version}",
+ domain=warehouse,
+ ),
pretend.call(
"manage.project.roles",
"/manage/project/{project_name}/collaboration/",
| Enable pages to delete, hide, unhide a project/release/file
We need UI that will allow users to modify the things they register and upload to Warehouse.
Add ability to delete release
The current UI allows users to do this via the releases page:
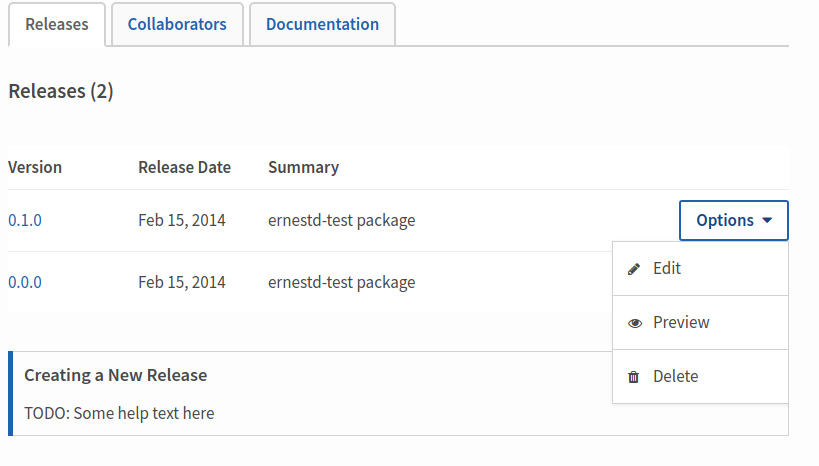
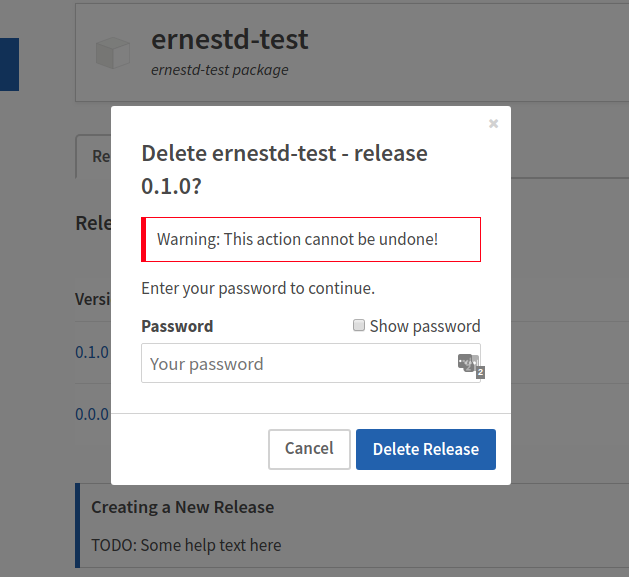
This code is currently commented out and should be enabled.
In addition, users should be able to delete a release via the release detail page (see https://github.com/pypa/warehouse/issues/2807)
| current layout:

How is this not implemented yet, when the legacy API no longer allows editing releases?
> Gone (This API has been deprecated and removed from legacy PyPI in favor of using the APIs available in the new PyPI.org implementation of PyPI (located at https://pypi.org/). For more information about migrating your use of this API to PyPI.org, please see https://packaging.python.org/guides/migrating-to-pypi-org/#uploading. For more information about the sunsetting of this API, please see https://mail.python.org/pipermail/distutils-sig/2017-June/030766.html)
There currently seems to be no way to edit a project/release/file on PyPi.org. How was the old API allowed to be shut-down when the new one lacks significant functionality?
In our [meeting today](https://wiki.python.org/psf/PackagingWG/2017-12-19-Warehouse) we discussed this issue and the larger question of release metadata immutability. Per @dstufft's [email to `distutils-sig`](https://mail.python.org/pipermail/distutils-sig/2017-December/031826.html) (reproduced in https://github.com/pypa/warehouse/issues/2170#issuecomment-352866597 ), we decided that we're going to narrow this issue to focus on the features we do want to implement:
* giving package managers a way to delete their own files, releases, and projects
* giving package maintainers a way to manage hidden vs unhidden releases
As I understand it, that set of features does belong in [the first milestone, the MVP for package maintainers](https://github.com/pypa/warehouse/milestone/8). @dstufft, @ewdurbin, @di and others, please correct me if I'm wrong.
As per @dstufft's comment here - https://github.com/pypa/warehouse/issues/2734#issuecomment-355788559 - we can remove "giving package maintainers a way to manage hidden vs unhidden releases" from this specification.
A sub ticket of #424 | 2018-02-02T18:06:52Z | [] | [] |
pypi/warehouse | 2,898 | pypi__warehouse-2898 | [
"2830"
] | b32ccb99fdefd8ece8e4b259ebefee276ae715c2 | diff --git a/warehouse/accounts/__init__.py b/warehouse/accounts/__init__.py
--- a/warehouse/accounts/__init__.py
+++ b/warehouse/accounts/__init__.py
@@ -75,6 +75,11 @@ def includeme(config):
ITokenService,
name="password",
)
+ config.register_service_factory(
+ TokenServiceFactory(name="email"),
+ ITokenService,
+ name="email",
+ )
# Register our authentication and authorization policies
config.set_authentication_policy(
diff --git a/warehouse/accounts/forms.py b/warehouse/accounts/forms.py
--- a/warehouse/accounts/forms.py
+++ b/warehouse/accounts/forms.py
@@ -100,9 +100,7 @@ class NewPasswordMixin:
)
-class RegistrationForm(NewPasswordMixin, NewUsernameMixin, forms.Form):
-
- full_name = wtforms.StringField()
+class NewEmailMixin:
email = wtforms.fields.html5.EmailField(
validators=[
@@ -116,13 +114,6 @@ class RegistrationForm(NewPasswordMixin, NewUsernameMixin, forms.Form):
],
)
- g_recaptcha_response = wtforms.StringField()
-
- def __init__(self, *args, recaptcha_service, user_service, **kwargs):
- super().__init__(*args, **kwargs)
- self.user_service = user_service
- self.recaptcha_service = recaptcha_service
-
def validate_email(self, field):
if self.user_service.find_userid_by_email(field.data) is not None:
raise wtforms.validators.ValidationError(
@@ -136,6 +127,18 @@ def validate_email(self, field):
"from this domain. Please use a different email."
)
+
+class RegistrationForm(
+ NewPasswordMixin, NewUsernameMixin, NewEmailMixin, forms.Form):
+
+ full_name = wtforms.StringField()
+ g_recaptcha_response = wtforms.StringField()
+
+ def __init__(self, *args, recaptcha_service, user_service, **kwargs):
+ super().__init__(*args, **kwargs)
+ self.user_service = user_service
+ self.recaptcha_service = recaptcha_service
+
def validate_g_recaptcha_response(self, field):
# do required data validation here due to enabled flag being required
if self.recaptcha_service.enabled and not field.data:
diff --git a/warehouse/accounts/interfaces.py b/warehouse/accounts/interfaces.py
--- a/warehouse/accounts/interfaces.py
+++ b/warehouse/accounts/interfaces.py
@@ -73,11 +73,6 @@ def update_user(user_id, **changes):
Updates the user object
"""
- def verify_email(user_id, email_address):
- """
- verifies the user
- """
-
class ITokenService(Interface):
diff --git a/warehouse/accounts/services.py b/warehouse/accounts/services.py
--- a/warehouse/accounts/services.py
+++ b/warehouse/accounts/services.py
@@ -173,12 +173,6 @@ def update_user(self, user_id, **changes):
setattr(user, attr, value)
return user
- def verify_email(self, user_id, email_address):
- user = self.get_user(user_id)
- for email in user.emails:
- if email.email == email_address:
- email.verified = True
-
@implementer(ITokenService)
class TokenService:
diff --git a/warehouse/accounts/views.py b/warehouse/accounts/views.py
--- a/warehouse/accounts/views.py
+++ b/warehouse/accounts/views.py
@@ -17,9 +17,10 @@
from pyramid.httpexceptions import (
HTTPMovedPermanently, HTTPSeeOther, HTTPTooManyRequests,
)
-from pyramid.security import remember, forget
+from pyramid.security import Authenticated, remember, forget
from pyramid.view import view_config
from sqlalchemy.orm import joinedload
+from sqlalchemy.orm.exc import NoResultFound
from warehouse.accounts import REDIRECT_FIELD_NAME
from warehouse.accounts.forms import (
@@ -29,6 +30,7 @@
IUserService, ITokenService, TokenExpired, TokenInvalid, TokenMissing,
TooManyFailedLogins,
)
+from warehouse.accounts.models import Email
from warehouse.cache.origin import origin_cache
from warehouse.email import send_password_reset_email
from warehouse.packaging.models import Project, Release
@@ -337,6 +339,55 @@ def _error(message):
return {"form": form}
+@view_config(
+ route_name="accounts.verify-email",
+ uses_session=True,
+ effective_principals=Authenticated,
+)
+def verify_email(request):
+ token_service = request.find_service(ITokenService, name="email")
+
+ def _error(message):
+ request.session.flash(message, queue="error")
+ return HTTPSeeOther(request.route_path("manage.profile"))
+
+ try:
+ token = request.params.get('token')
+ data = token_service.loads(token)
+ except TokenExpired:
+ return _error("Expired token - Request a new verification link")
+ except TokenInvalid:
+ return _error("Invalid token - Request a new verification link")
+ except TokenMissing:
+ return _error("Invalid token - No token supplied")
+
+ # Check whether this token is being used correctly
+ if data.get('action') != "email-verify":
+ return _error("Invalid token - Not an email verification token")
+
+ try:
+ email = (
+ request.db.query(Email)
+ .filter(Email.id == data['email.id'], Email.user == request.user)
+ .one()
+ )
+ except NoResultFound:
+ return _error("Email not found")
+
+ if email.verified:
+ return _error("Email already verified")
+
+ email.verified = True
+ request.user.is_active = True
+
+ request.session.flash(
+ f'Email address {email.email} verified. ' +
+ 'You can now set this email as your primary address.',
+ queue='success'
+ )
+ return HTTPSeeOther(request.route_path("manage.profile"))
+
+
def _login_user(request, userid):
# We have a session factory associated with this request, so in order
# to protect against session fixation attacks we're going to make sure
diff --git a/warehouse/config.py b/warehouse/config.py
--- a/warehouse/config.py
+++ b/warehouse/config.py
@@ -173,12 +173,19 @@ def configure(settings=None):
maybe_set(settings, "ga.tracking_id", "GA_TRACKING_ID")
maybe_set(settings, "statuspage.url", "STATUSPAGE_URL")
maybe_set(settings, "token.password.secret", "TOKEN_PASSWORD_SECRET")
+ maybe_set(settings, "token.email.secret", "TOKEN_EMAIL_SECRET")
maybe_set(
settings,
"token.password.max_age",
"TOKEN_PASSWORD_MAX_AGE",
coercer=int,
)
+ maybe_set(
+ settings,
+ "token.email.max_age",
+ "TOKEN_EMAIL_MAX_AGE",
+ coercer=int,
+ )
maybe_set(
settings,
"token.default.max_age",
diff --git a/warehouse/email.py b/warehouse/email.py
--- a/warehouse/email.py
+++ b/warehouse/email.py
@@ -67,3 +67,34 @@ def send_password_reset_email(request, user):
# Return the fields we used, in case we need to show any of them to the
# user
return fields
+
+
+def send_email_verification_email(request, email):
+ token_service = request.find_service(ITokenService, name='email')
+
+ token = token_service.dumps({
+ "action": "email-verify",
+ "email.id": email.id,
+ })
+
+ fields = {
+ 'token': token,
+ 'email_address': email.email,
+ 'n_hours': token_service.max_age // 60 // 60,
+ }
+
+ subject = render(
+ 'email/verify-email.subject.txt',
+ fields,
+ request=request,
+ )
+
+ body = render(
+ 'email/verify-email.body.txt',
+ fields,
+ request=request,
+ )
+
+ request.task(send_email).delay(body, [email.email], subject)
+
+ return fields
diff --git a/warehouse/manage/forms.py b/warehouse/manage/forms.py
--- a/warehouse/manage/forms.py
+++ b/warehouse/manage/forms.py
@@ -13,6 +13,7 @@
import wtforms
from warehouse import forms
+from warehouse.accounts.forms import NewEmailMixin
class RoleNameMixin:
@@ -62,3 +63,12 @@ class SaveProfileForm(forms.Form):
__params__ = ['name']
name = wtforms.StringField()
+
+
+class AddEmailForm(NewEmailMixin, forms.Form):
+
+ __params__ = ['email']
+
+ def __init__(self, *args, user_service, **kwargs):
+ super().__init__(*args, **kwargs)
+ self.user_service = user_service
diff --git a/warehouse/manage/views.py b/warehouse/manage/views.py
--- a/warehouse/manage/views.py
+++ b/warehouse/manage/views.py
@@ -19,9 +19,10 @@
from sqlalchemy.orm.exc import NoResultFound
from warehouse.accounts.interfaces import IUserService
-from warehouse.accounts.models import User
+from warehouse.accounts.models import User, Email
+from warehouse.email import send_email_verification_email
from warehouse.manage.forms import (
- CreateRoleForm, ChangeRoleForm, SaveProfileForm
+ AddEmailForm, CreateRoleForm, ChangeRoleForm, SaveProfileForm
)
from warehouse.packaging.models import JournalEntry, Role, File
from warehouse.utils.project import confirm_project, remove_project
@@ -40,12 +41,17 @@ def __init__(self, request):
self.request = request
self.user_service = request.find_service(IUserService, context=None)
- @view_config(request_method="GET")
- def manage_profile(self):
+ @property
+ def default_response(self):
return {
'save_profile_form': SaveProfileForm(name=self.request.user.name),
+ 'add_email_form': AddEmailForm(user_service=self.user_service),
}
+ @view_config(request_method="GET")
+ def manage_profile(self):
+ return self.default_response
+
@view_config(
request_method="POST",
request_param=SaveProfileForm.__params__,
@@ -60,9 +66,129 @@ def save_profile(self):
)
return {
+ **self.default_response,
'save_profile_form': form,
}
+ @view_config(
+ request_method="POST",
+ request_param=AddEmailForm.__params__,
+ )
+ def add_email(self):
+ form = AddEmailForm(self.request.POST, user_service=self.user_service)
+
+ if form.validate():
+ email = Email(
+ email=form.email.data,
+ user_id=self.request.user.id,
+ primary=False,
+ verified=False,
+ )
+ self.request.user.emails.append(email)
+ self.request.db.flush() # To get the new ID
+
+ send_email_verification_email(self.request, email)
+
+ self.request.session.flash(
+ f'Email {email.email} added - check your email for ' +
+ 'a verification link.',
+ queue='success',
+ )
+ return self.default_response
+
+ return {
+ **self.default_response,
+ 'add_email_form': form,
+ }
+
+ @view_config(
+ request_method="POST",
+ request_param=["delete_email_id"],
+ )
+ def delete_email(self):
+ try:
+ email = self.request.db.query(Email).filter(
+ Email.id == self.request.POST['delete_email_id'],
+ Email.user_id == self.request.user.id,
+ ).one()
+ except NoResultFound:
+ self.request.session.flash(
+ 'Email address not found.', queue='error'
+ )
+ return self.default_response
+
+ if email.primary:
+ self.request.session.flash(
+ 'Cannot remove primary email address.', queue='error'
+ )
+ else:
+ self.request.user.emails.remove(email)
+ self.request.session.flash(
+ f'Email address {email.email} removed.', queue='success'
+ )
+ return self.default_response
+
+ @view_config(
+ request_method="POST",
+ request_param=["primary_email_id"],
+ )
+ def change_primary_email(self):
+ try:
+ new_primary_email = self.request.db.query(Email).filter(
+ Email.user_id == self.request.user.id,
+ Email.id == self.request.POST['primary_email_id'],
+ Email.verified.is_(True),
+ ).one()
+ except NoResultFound:
+ self.request.session.flash(
+ 'Email address not found.', queue='error'
+ )
+ return self.default_response
+
+ self.request.db.query(Email).filter(
+ Email.user_id == self.request.user.id,
+ Email.primary.is_(True),
+ ).update(values={'primary': False})
+
+ new_primary_email.primary = True
+
+ self.request.session.flash(
+ f'Email address {new_primary_email.email} set as primary.',
+ queue='success',
+ )
+
+ return self.default_response
+
+ @view_config(
+ request_method="POST",
+ request_param=['reverify_email_id'],
+ )
+ def reverify_email(self):
+ try:
+ email = self.request.db.query(Email).filter(
+ Email.id == self.request.POST['reverify_email_id'],
+ Email.user_id == self.request.user.id,
+ ).one()
+ except NoResultFound:
+ self.request.session.flash(
+ 'Email address not found.', queue='error'
+ )
+ return self.default_response
+
+ if email.verified:
+ self.request.session.flash(
+ 'Email is already verified.', queue='error'
+ )
+ else:
+ send_email_verification_email(self.request, email)
+
+ self.request.session.flash(
+ f'Verification email for {email.email} resent.',
+ queue='success',
+ )
+
+ return self.default_response
+
@view_config(
route_name="manage.projects",
diff --git a/warehouse/routes.py b/warehouse/routes.py
--- a/warehouse/routes.py
+++ b/warehouse/routes.py
@@ -109,6 +109,11 @@ def includeme(config):
"/account/reset-password/",
domain=warehouse,
)
+ config.add_route(
+ "accounts.verify-email",
+ "/account/verify-email/",
+ domain=warehouse,
+ )
# Management (views for logged-in users)
config.add_route("manage.profile", "/manage/profile/", domain=warehouse)
| diff --git a/tests/common/db/accounts.py b/tests/common/db/accounts.py
--- a/tests/common/db/accounts.py
+++ b/tests/common/db/accounts.py
@@ -46,3 +46,4 @@ class Meta:
user = factory.SubFactory(UserFactory)
email = FuzzyEmail()
verified = True
+ primary = True
diff --git a/tests/unit/accounts/test_services.py b/tests/unit/accounts/test_services.py
--- a/tests/unit/accounts/test_services.py
+++ b/tests/unit/accounts/test_services.py
@@ -162,16 +162,6 @@ def test_update_user(self, user_service):
assert password != user_from_db.password
assert user_service.hasher.verify(password, user_from_db.password)
- def test_verify_email(self, user_service):
- user = UserFactory.create()
- EmailFactory.create(user=user, primary=True,
- verified=False)
- EmailFactory.create(user=user, primary=False,
- verified=False)
- user_service.verify_email(user.id, user.emails[0].email)
- assert user.emails[0].verified
- assert not user.emails[1].verified
-
def test_find_by_email(self, user_service):
user = UserFactory.create()
EmailFactory.create(user=user, primary=True, verified=False)
diff --git a/tests/unit/accounts/test_views.py b/tests/unit/accounts/test_views.py
--- a/tests/unit/accounts/test_views.py
+++ b/tests/unit/accounts/test_views.py
@@ -18,6 +18,7 @@
import pytest
from pyramid.httpexceptions import HTTPMovedPermanently, HTTPSeeOther
+from sqlalchemy.orm.exc import NoResultFound
from warehouse.accounts import views
from warehouse.accounts.interfaces import (
@@ -25,7 +26,7 @@
TooManyFailedLogins
)
-from ...common.db.accounts import UserFactory
+from ...common.db.accounts import EmailFactory, UserFactory
class TestFailedLoginView:
@@ -695,6 +696,166 @@ def test_reset_password_password_date_changed(self, pyramid_request):
]
+class TestVerifyEmail:
+
+ def test_verify_email(self, db_request, user_service, token_service):
+ user = UserFactory(is_active=False)
+ email = EmailFactory(user=user, verified=False)
+ db_request.user = user
+ db_request.GET.update({"token": "RANDOM_KEY"})
+ db_request.route_path = pretend.call_recorder(lambda name: "/")
+ token_service.loads = pretend.call_recorder(
+ lambda token: {
+ 'action': 'email-verify',
+ 'email.id': str(email.id),
+ }
+ )
+ db_request.find_service = pretend.call_recorder(
+ lambda *a, **kwargs: token_service,
+ )
+ db_request.session.flash = pretend.call_recorder(
+ lambda *a, **kw: None
+ )
+
+ result = views.verify_email(db_request)
+
+ db_request.db.flush()
+ assert email.verified
+ assert user.is_active
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/"
+ assert db_request.route_path.calls == [pretend.call('manage.profile')]
+ assert token_service.loads.calls == [pretend.call('RANDOM_KEY')]
+ assert db_request.session.flash.calls == [
+ pretend.call(
+ f"Email address {email.email} verified. " +
+ "You can now set this email as your primary address.",
+ queue="success"
+ ),
+ ]
+ assert db_request.find_service.calls == [
+ pretend.call(ITokenService, name="email"),
+ ]
+
+ @pytest.mark.parametrize(
+ ("exception", "message"),
+ [
+ (
+ TokenInvalid,
+ "Invalid token - Request a new verification link",
+ ), (
+ TokenExpired,
+ "Expired token - Request a new verification link",
+ ), (
+ TokenMissing,
+ "Invalid token - No token supplied"
+ ),
+ ],
+ )
+ def test_verify_email_loads_failure(
+ self, pyramid_request, exception, message):
+
+ def loads(token):
+ raise exception
+
+ pyramid_request.find_service = (
+ lambda *a, **kw: pretend.stub(loads=loads)
+ )
+ pyramid_request.params = {"token": "RANDOM_KEY"}
+ pyramid_request.route_path = pretend.call_recorder(lambda name: "/")
+ pyramid_request.session.flash = pretend.call_recorder(
+ lambda *a, **kw: None
+ )
+
+ views.verify_email(pyramid_request)
+
+ assert pyramid_request.route_path.calls == [
+ pretend.call('manage.profile'),
+ ]
+ assert pyramid_request.session.flash.calls == [
+ pretend.call(message, queue='error'),
+ ]
+
+ def test_verify_email_invalid_action(self, pyramid_request):
+ data = {
+ 'action': 'invalid-action',
+ }
+ pyramid_request.find_service = (
+ lambda *a, **kw: pretend.stub(loads=lambda a: data)
+ )
+ pyramid_request.params = {"token": "RANDOM_KEY"}
+ pyramid_request.route_path = pretend.call_recorder(lambda name: "/")
+ pyramid_request.session.flash = pretend.call_recorder(
+ lambda *a, **kw: None
+ )
+
+ views.verify_email(pyramid_request)
+
+ assert pyramid_request.route_path.calls == [
+ pretend.call('manage.profile'),
+ ]
+ assert pyramid_request.session.flash.calls == [
+ pretend.call(
+ "Invalid token - Not an email verification token",
+ queue='error',
+ ),
+ ]
+
+ def test_verify_email_not_found(self, pyramid_request):
+ data = {
+ 'action': 'email-verify',
+ 'email.id': 'invalid',
+ }
+ pyramid_request.find_service = (
+ lambda *a, **kw: pretend.stub(loads=lambda a: data)
+ )
+ pyramid_request.params = {"token": "RANDOM_KEY"}
+ pyramid_request.route_path = pretend.call_recorder(lambda name: "/")
+ pyramid_request.session.flash = pretend.call_recorder(
+ lambda *a, **kw: None
+ )
+
+ def raise_no_result(*a):
+ raise NoResultFound
+
+ pyramid_request.db = pretend.stub(query=raise_no_result)
+
+ views.verify_email(pyramid_request)
+
+ assert pyramid_request.route_path.calls == [
+ pretend.call('manage.profile'),
+ ]
+ assert pyramid_request.session.flash.calls == [
+ pretend.call('Email not found', queue='error')
+ ]
+
+ def test_verify_email_already_verified(self, db_request):
+ user = UserFactory()
+ email = EmailFactory(user=user, verified=True)
+ data = {
+ 'action': 'email-verify',
+ 'email.id': email.id,
+ }
+ db_request.user = user
+ db_request.find_service = (
+ lambda *a, **kw: pretend.stub(loads=lambda a: data)
+ )
+ db_request.params = {"token": "RANDOM_KEY"}
+ db_request.route_path = pretend.call_recorder(lambda name: "/")
+ db_request.session.flash = pretend.call_recorder(
+ lambda *a, **kw: None
+ )
+
+ views.verify_email(db_request)
+
+ assert db_request.route_path.calls == [
+ pretend.call('manage.profile'),
+ ]
+ assert db_request.session.flash.calls == [
+ pretend.call('Email already verified', queue='error')
+ ]
+
+
class TestProfileCallout:
def test_profile_callout_returns_user(self):
diff --git a/tests/unit/manage/test_forms.py b/tests/unit/manage/test_forms.py
--- a/tests/unit/manage/test_forms.py
+++ b/tests/unit/manage/test_forms.py
@@ -69,3 +69,12 @@ def test_validate_role_name_fails(self, value, expected):
assert not form.validate()
assert form.role_name.errors == [expected]
+
+
+class TestAddEmailForm:
+
+ def test_creation(self):
+ user_service = pretend.stub()
+ form = forms.AddEmailForm(user_service=user_service)
+
+ assert form.user_service is user_service
diff --git a/tests/unit/manage/test_views.py b/tests/unit/manage/test_views.py
--- a/tests/unit/manage/test_views.py
+++ b/tests/unit/manage/test_views.py
@@ -24,6 +24,7 @@
from warehouse.accounts.interfaces import IUserService
from warehouse.packaging.models import JournalEntry, Project, Role
+from ...common.db.accounts import EmailFactory
from ...common.db.packaging import (
JournalEntryFactory, ProjectFactory, RoleFactory, UserFactory,
)
@@ -31,7 +32,7 @@
class TestManageProfile:
- def test_manage_profile(self, monkeypatch):
+ def test_default_response(self, monkeypatch):
user_service = pretend.stub()
name = pretend.stub()
request = pretend.stub(
@@ -41,38 +42,64 @@ def test_manage_profile(self, monkeypatch):
save_profile_obj = pretend.stub()
save_profile_cls = pretend.call_recorder(lambda **kw: save_profile_obj)
monkeypatch.setattr(views, 'SaveProfileForm', save_profile_cls)
- view_class = views.ManageProfileViews(request)
+ add_email_obj = pretend.stub()
+ add_email_cls = pretend.call_recorder(lambda *a, **kw: add_email_obj)
+ monkeypatch.setattr(views, 'AddEmailForm', add_email_cls)
+ view = views.ManageProfileViews(request)
- assert view_class.manage_profile() == {
+ assert view.default_response == {
'save_profile_form': save_profile_obj,
+ 'add_email_form': add_email_obj,
}
- assert view_class.request == request
- assert view_class.user_service == user_service
+ assert view.request == request
+ assert view.user_service == user_service
assert save_profile_cls.calls == [
pretend.call(name=name),
]
+ assert add_email_cls.calls == [
+ pretend.call(user_service=user_service),
+ ]
+
+ def test_manage_profile(self, monkeypatch):
+ user_service = pretend.stub()
+ name = pretend.stub()
+ request = pretend.stub(
+ find_service=lambda *a, **kw: user_service,
+ user=pretend.stub(name=name),
+ )
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'default_response', {'_': pretend.stub()}
+ )
+ view = views.ManageProfileViews(request)
+
+ assert view.manage_profile() == view.default_response
+ assert view.request == request
+ assert view.user_service == user_service
def test_save_profile(self, monkeypatch):
update_user = pretend.call_recorder(lambda *a, **kw: None)
+ user_service = pretend.stub(update_user=update_user)
request = pretend.stub(
POST={'name': 'new name'},
- user=pretend.stub(id=pretend.stub()),
+ user=pretend.stub(id=pretend.stub(), name=pretend.stub()),
session=pretend.stub(
flash=pretend.call_recorder(lambda *a, **kw: None),
),
- find_service=pretend.call_recorder(
- lambda iface, context: pretend.stub(update_user=update_user)
- ),
+ find_service=lambda *a, **kw: user_service,
)
save_profile_obj = pretend.stub(
- validate=lambda: True,
- data=request.POST,
+ validate=lambda: True, data=request.POST
)
- save_profile_cls = pretend.call_recorder(lambda d: save_profile_obj)
- monkeypatch.setattr(views, 'SaveProfileForm', save_profile_cls)
- view_class = views.ManageProfileViews(request)
+ monkeypatch.setattr(
+ views, 'SaveProfileForm', lambda *a, **kw: save_profile_obj
+ )
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'default_response', {'_': pretend.stub()}
+ )
+ view = views.ManageProfileViews(request)
- assert view_class.save_profile() == {
+ assert view.save_profile() == {
+ **view.default_response,
'save_profile_form': save_profile_obj,
}
assert request.session.flash.calls == [
@@ -81,35 +108,360 @@ def test_save_profile(self, monkeypatch):
assert update_user.calls == [
pretend.call(request.user.id, **request.POST)
]
- assert save_profile_cls.calls == [
- pretend.call(request.POST),
- ]
def test_save_profile_validation_fails(self, monkeypatch):
update_user = pretend.call_recorder(lambda *a, **kw: None)
+ user_service = pretend.stub(update_user=update_user)
request = pretend.stub(
POST={'name': 'new name'},
- user=pretend.stub(id=pretend.stub()),
+ user=pretend.stub(id=pretend.stub(), name=pretend.stub()),
session=pretend.stub(
flash=pretend.call_recorder(lambda *a, **kw: None),
),
- find_service=pretend.call_recorder(
- lambda iface, context: pretend.stub(update_user=update_user)
- ),
+ find_service=lambda *a, **kw: user_service,
)
save_profile_obj = pretend.stub(validate=lambda: False)
- save_profile_cls = pretend.call_recorder(lambda d: save_profile_obj)
- monkeypatch.setattr(views, 'SaveProfileForm', save_profile_cls)
- view_class = views.ManageProfileViews(request)
+ monkeypatch.setattr(
+ views, 'SaveProfileForm', lambda *a, **kw: save_profile_obj
+ )
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'default_response', {'_': pretend.stub()}
+ )
+ view = views.ManageProfileViews(request)
- assert view_class.save_profile() == {
+ assert view.save_profile() == {
+ **view.default_response,
'save_profile_form': save_profile_obj,
}
assert request.session.flash.calls == []
assert update_user.calls == []
- assert save_profile_cls.calls == [
- pretend.call(request.POST),
+
+ def test_add_email(self, monkeypatch, pyramid_config):
+ email_address = "[email protected]"
+ user_service = pretend.stub()
+ request = pretend.stub(
+ POST={'email': email_address},
+ db=pretend.stub(flush=lambda: None),
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ ),
+ find_service=lambda a, **kw: user_service,
+ user=pretend.stub(
+ emails=[], name=pretend.stub(), id=pretend.stub()
+ ),
+ task=pretend.call_recorder(lambda *args, **kwargs: send_email),
+ )
+ monkeypatch.setattr(
+ views, 'AddEmailForm', lambda *a, **kw: pretend.stub(
+ validate=lambda: True,
+ email=pretend.stub(data=email_address),
+ )
+ )
+
+ email_obj = pretend.stub(id=pretend.stub(), email=email_address)
+ email_cls = pretend.call_recorder(lambda **kw: email_obj)
+ monkeypatch.setattr(views, 'Email', email_cls)
+
+ send_email = pretend.call_recorder(lambda *a: None)
+ monkeypatch.setattr(views, 'send_email_verification_email', send_email)
+
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'default_response', {'_': pretend.stub()}
+ )
+ view = views.ManageProfileViews(request)
+
+ assert view.add_email() == view.default_response
+ assert request.user.emails == [email_obj]
+ assert email_cls.calls == [
+ pretend.call(
+ email=email_address,
+ user_id=request.user.id,
+ primary=False,
+ verified=False,
+ ),
+ ]
+ assert request.session.flash.calls == [
+ pretend.call(
+ f'Email {email_address} added - check your email for ' +
+ 'a verification link.',
+ queue='success',
+ ),
+ ]
+ assert send_email.calls == [
+ pretend.call(request, email_obj),
+ ]
+
+ def test_add_email_validation_fails(self, monkeypatch):
+ email_address = "[email protected]"
+ request = pretend.stub(
+ POST={'email': email_address},
+ db=pretend.stub(flush=lambda: None),
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ ),
+ find_service=lambda a, **kw: pretend.stub(),
+ user=pretend.stub(emails=[], name=pretend.stub()),
+ )
+ add_email_obj = pretend.stub(
+ validate=lambda: False,
+ email=pretend.stub(data=email_address),
+ )
+ add_email_cls = pretend.call_recorder(lambda *a, **kw: add_email_obj)
+ monkeypatch.setattr(views, 'AddEmailForm', add_email_cls)
+
+ email_obj = pretend.stub(id=pretend.stub(), email=email_address)
+ email_cls = pretend.call_recorder(lambda **kw: email_obj)
+ monkeypatch.setattr(views, 'Email', email_cls)
+
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'default_response', {'_': pretend.stub()}
+ )
+ view = views.ManageProfileViews(request)
+
+ assert view.add_email() == {
+ **view.default_response,
+ 'add_email_form': add_email_obj,
+ }
+ assert request.user.emails == []
+ assert email_cls.calls == []
+ assert request.session.flash.calls == []
+
+ def test_delete_email(self, monkeypatch):
+ email = pretend.stub(
+ id=pretend.stub(), primary=False, email=pretend.stub(),
+ )
+ some_other_email = pretend.stub()
+ request = pretend.stub(
+ POST={'delete_email_id': email.id},
+ user=pretend.stub(
+ id=pretend.stub(),
+ emails=[email, some_other_email],
+ name=pretend.stub(),
+ ),
+ db=pretend.stub(
+ query=lambda a: pretend.stub(
+ filter=lambda *a: pretend.stub(one=lambda: email)
+ ),
+ ),
+ find_service=lambda *a, **kw: pretend.stub(),
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ )
+ )
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'default_response', {'_': pretend.stub()}
+ )
+ view = views.ManageProfileViews(request)
+
+ assert view.delete_email() == view.default_response
+ assert request.session.flash.calls == [
+ pretend.call(
+ f'Email address {email.email} removed.', queue='success'
+ )
+ ]
+ assert request.user.emails == [some_other_email]
+
+ def test_delete_email_not_found(self, monkeypatch):
+ email = pretend.stub()
+
+ def raise_no_result():
+ raise NoResultFound
+
+ request = pretend.stub(
+ POST={'delete_email_id': 'missing_id'},
+ user=pretend.stub(
+ id=pretend.stub(),
+ emails=[email],
+ name=pretend.stub(),
+ ),
+ db=pretend.stub(
+ query=lambda a: pretend.stub(
+ filter=lambda *a: pretend.stub(one=raise_no_result)
+ ),
+ ),
+ find_service=lambda *a, **kw: pretend.stub(),
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ )
+ )
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'default_response', {'_': pretend.stub()}
+ )
+ view = views.ManageProfileViews(request)
+
+ assert view.delete_email() == view.default_response
+ assert request.session.flash.calls == [
+ pretend.call('Email address not found.', queue='error'),
+ ]
+ assert request.user.emails == [email]
+
+ def test_delete_email_is_primary(self, monkeypatch):
+ email = pretend.stub(primary=True)
+
+ request = pretend.stub(
+ POST={'delete_email_id': 'missing_id'},
+ user=pretend.stub(
+ id=pretend.stub(),
+ emails=[email],
+ name=pretend.stub(),
+ ),
+ db=pretend.stub(
+ query=lambda a: pretend.stub(
+ filter=lambda *a: pretend.stub(one=lambda: email)
+ ),
+ ),
+ find_service=lambda *a, **kw: pretend.stub(),
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ )
+ )
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'default_response', {'_': pretend.stub()}
+ )
+ view = views.ManageProfileViews(request)
+
+ assert view.delete_email() == view.default_response
+ assert request.session.flash.calls == [
+ pretend.call(
+ 'Cannot remove primary email address.', queue='error'
+ ),
+ ]
+ assert request.user.emails == [email]
+
+ def test_change_primary_email(self, monkeypatch, db_request):
+ user = UserFactory()
+ old_primary = EmailFactory(primary=True, user=user)
+ new_primary = EmailFactory(primary=False, verified=True, user=user)
+
+ db_request.user = user
+ db_request.find_service = lambda *a, **kw: pretend.stub()
+ db_request.POST = {'primary_email_id': new_primary.id}
+ db_request.session.flash = pretend.call_recorder(lambda *a, **kw: None)
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'default_response', {'_': pretend.stub()}
+ )
+ view = views.ManageProfileViews(db_request)
+
+ assert view.change_primary_email() == view.default_response
+ assert db_request.session.flash.calls == [
+ pretend.call(
+ f'Email address {new_primary.email} set as primary.',
+ queue='success',
+ )
+ ]
+ assert not old_primary.primary
+ assert new_primary.primary
+
+ def test_change_primary_email_not_found(self, monkeypatch, db_request):
+ user = UserFactory()
+ old_primary = EmailFactory(primary=True, user=user)
+ missing_email_id = 9999
+
+ db_request.user = user
+ db_request.find_service = lambda *a, **kw: pretend.stub()
+ db_request.POST = {'primary_email_id': missing_email_id}
+ db_request.session.flash = pretend.call_recorder(lambda *a, **kw: None)
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'default_response', {'_': pretend.stub()}
+ )
+ view = views.ManageProfileViews(db_request)
+
+ assert view.change_primary_email() == view.default_response
+ assert db_request.session.flash.calls == [
+ pretend.call(f'Email address not found.', queue='error')
+ ]
+ assert old_primary.primary
+
+ def test_reverify_email(self, monkeypatch):
+ email = pretend.stub(verified=False, email='email_address')
+
+ request = pretend.stub(
+ POST={'reverify_email_id': pretend.stub()},
+ db=pretend.stub(
+ query=lambda *a: pretend.stub(
+ filter=lambda *a: pretend.stub(one=lambda: email)
+ ),
+ ),
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None)
+ ),
+ find_service=lambda *a, **kw: pretend.stub(),
+ user=pretend.stub(id=pretend.stub),
+ )
+ send_email = pretend.call_recorder(lambda *a: None)
+ monkeypatch.setattr(views, 'send_email_verification_email', send_email)
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'default_response', {'_': pretend.stub()}
+ )
+ view = views.ManageProfileViews(request)
+
+ assert view.reverify_email() == view.default_response
+ assert request.session.flash.calls == [
+ pretend.call(
+ 'Verification email for email_address resent.',
+ queue='success',
+ ),
+ ]
+ assert send_email.calls == [pretend.call(request, email)]
+
+ def test_reverify_email_not_found(self, monkeypatch):
+ def raise_no_result():
+ raise NoResultFound
+
+ request = pretend.stub(
+ POST={'reverify_email_id': pretend.stub()},
+ db=pretend.stub(
+ query=lambda *a: pretend.stub(
+ filter=lambda *a: pretend.stub(one=raise_no_result)
+ ),
+ ),
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None)
+ ),
+ find_service=lambda *a, **kw: pretend.stub(),
+ user=pretend.stub(id=pretend.stub),
+ )
+ send_email = pretend.call_recorder(lambda *a: None)
+ monkeypatch.setattr(views, 'send_email_verification_email', send_email)
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'default_response', {'_': pretend.stub()}
+ )
+ view = views.ManageProfileViews(request)
+
+ assert view.reverify_email() == view.default_response
+ assert request.session.flash.calls == [
+ pretend.call('Email address not found.', queue='error'),
+ ]
+ assert send_email.calls == []
+
+ def test_reverify_email_already_verified(self, monkeypatch):
+ email = pretend.stub(verified=True, email='email_address')
+
+ request = pretend.stub(
+ POST={'reverify_email_id': pretend.stub()},
+ db=pretend.stub(
+ query=lambda *a: pretend.stub(
+ filter=lambda *a: pretend.stub(one=lambda: email)
+ ),
+ ),
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None)
+ ),
+ find_service=lambda *a, **kw: pretend.stub(),
+ user=pretend.stub(id=pretend.stub),
+ )
+ send_email = pretend.call_recorder(lambda *a: None)
+ monkeypatch.setattr(views, 'send_email_verification_email', send_email)
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'default_response', {'_': pretend.stub()}
+ )
+ view = views.ManageProfileViews(request)
+
+ assert view.reverify_email() == view.default_response
+ assert request.session.flash.calls == [
+ pretend.call('Email is already verified.', queue='error'),
]
+ assert send_email.calls == []
class TestManageProjects:
diff --git a/tests/unit/test_email.py b/tests/unit/test_email.py
--- a/tests/unit/test_email.py
+++ b/tests/unit/test_email.py
@@ -156,3 +156,64 @@ def test_send_password_reset_email(
assert send_email.delay.calls == [
pretend.call('Email Body', [stub_user.email], 'Email Subject'),
]
+
+
+class TestEmailVerificationEmail:
+
+ def test_email_verification_email(
+ self, pyramid_request, pyramid_config, token_service, monkeypatch):
+
+ stub_email = pretend.stub(
+ id='id',
+ email='email',
+ )
+ pyramid_request.method = 'POST'
+ token_service.dumps = pretend.call_recorder(lambda a: 'TOKEN')
+ pyramid_request.find_service = pretend.call_recorder(
+ lambda *a, **kw: token_service
+ )
+
+ subject_renderer = pyramid_config.testing_add_renderer(
+ 'email/verify-email.subject.txt'
+ )
+ subject_renderer.string_response = 'Email Subject'
+ body_renderer = pyramid_config.testing_add_renderer(
+ 'email/verify-email.body.txt'
+ )
+ body_renderer.string_response = 'Email Body'
+
+ send_email = pretend.stub(
+ delay=pretend.call_recorder(lambda *args, **kwargs: None)
+ )
+ pyramid_request.task = pretend.call_recorder(
+ lambda *args, **kwargs: send_email
+ )
+ monkeypatch.setattr(email, 'send_email', send_email)
+
+ result = email.send_email_verification_email(
+ pyramid_request,
+ email=stub_email,
+ )
+
+ assert result == {
+ 'token': 'TOKEN',
+ 'email_address': stub_email.email,
+ 'n_hours': token_service.max_age // 60 // 60,
+ }
+ subject_renderer.assert_()
+ body_renderer.assert_(token='TOKEN', email_address=stub_email.email)
+ assert token_service.dumps.calls == [
+ pretend.call({
+ 'action': 'email-verify',
+ 'email.id': str(stub_email.id),
+ }),
+ ]
+ assert pyramid_request.find_service.calls == [
+ pretend.call(ITokenService, name='email'),
+ ]
+ assert pyramid_request.task.calls == [
+ pretend.call(send_email),
+ ]
+ assert send_email.delay.calls == [
+ pretend.call('Email Body', [stub_email.email], 'Email Subject'),
+ ]
diff --git a/tests/unit/test_routes.py b/tests/unit/test_routes.py
--- a/tests/unit/test_routes.py
+++ b/tests/unit/test_routes.py
@@ -137,6 +137,11 @@ def add_policy(name, filename):
"/account/reset-password/",
domain=warehouse,
),
+ pretend.call(
+ "accounts.verify-email",
+ "/account/verify-email/",
+ domain=warehouse,
+ ),
pretend.call(
"manage.profile",
"/manage/profile/",
| Manage Account: Change emails
From #423: From the 'manage account' page, a logged-in user should be able to do the following:
* view all emails associated with their account
* including the verified/unverified and/or primary status of each email address
* add a new email address
* should send a verification email to that address.
* change primary email address
| 2018-02-07T00:17:36Z | [] | [] |
|
pypi/warehouse | 2,904 | pypi__warehouse-2904 | [
"2679"
] | 27c610d5a801358360fdad46b1f12998229f5f4d | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -10,6 +10,7 @@
# See the License for the specific language governing permissions and
# limitations under the License.
+import email
import hashlib
import hmac
import os.path
@@ -255,6 +256,14 @@ def _validate_project_url_list(form, field):
_validate_project_url(datum)
+def _validate_rfc822_email_field(form, field):
+ email_validator = wtforms.validators.Email(message='Invalid email address')
+ addresses = email.utils.getaddresses([field.data])
+
+ for real_name, address in addresses:
+ email_validator(form, type('field', (), {'data': address}))
+
+
def _construct_dependencies(form, types):
for name, kind in types.items():
for item in getattr(form, name).data:
@@ -338,7 +347,7 @@ class MetadataForm(forms.Form):
description="Author-email",
validators=[
wtforms.validators.Optional(),
- wtforms.validators.Email(),
+ _validate_rfc822_email_field,
],
)
maintainer = wtforms.StringField(
@@ -349,7 +358,7 @@ class MetadataForm(forms.Form):
description="Maintainer-email",
validators=[
wtforms.validators.Optional(),
- wtforms.validators.Email(),
+ _validate_rfc822_email_field,
],
)
license = wtforms.StringField(
| diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -266,6 +266,37 @@ def test_validate_project_url_list(self, monkeypatch):
assert validator.calls == [pretend.call(datum) for datum in data]
+ @pytest.mark.parametrize(
+ 'data',
+ [
+ (''),
+ ('[email protected]'),
+ ('[email protected],'),
+ ('[email protected], [email protected]'),
+ ('"C. Schultz" <[email protected]>'),
+ ('"C. Schultz" <[email protected]>, [email protected]'),
+ ]
+ )
+ def test_validate_rfc822_email_field(self, data):
+ form, field = pretend.stub(), pretend.stub(data=data)
+ legacy._validate_rfc822_email_field(form, field)
+
+ @pytest.mark.parametrize(
+ 'data',
+ [
+ ('foo'),
+ ('foo@'),
+ ('@bar.com'),
+ ('foo@bar'),
+ ('foo AT bar DOT com'),
+ ('[email protected], foo'),
+ ]
+ )
+ def test_validate_rfc822_email_field_raises(self, data):
+ form, field = pretend.stub(), pretend.stub(data=data)
+ with pytest.raises(ValidationError):
+ legacy._validate_rfc822_email_field(form, field)
+
def test_construct_dependencies():
types = {
| Valid `Author-email` and `Maintainer-email` fields are rejected
Per https://packaging.python.org/specifications/core-metadata/#author-email-optional:
> A string containing the author’s e-mail address. It can contain a name and e-mail address in the legal forms for a RFC-822 `From:` header
>
> Example:
>
> `Author-email: "C. Schultz" <[email protected]>`
However if the provided `Author-email` field is set to the example value, the resulting distribution is rejected:
```
HTTPError: 400 Client Error: author_email: Invalid email address. for url: http://warehouse.local/legacy/
```
Because we're validating that this field is an email address, not a valid RFC-822 style header.
| I am willing to take a shot at this. Would it be fine if I ask my possibly dumb questions here?
@pradyunsg no question is a dumb question :)
Yes, If you have any questions, please ask here - we will do our best to help you.
Just wanted to point out to any potential new contributors that @pradyunsg hasn't submitted a PR for this issue (yet!) so it's still up for grabs.
I am not sure that the definition of the format matches the data model of the Metadata PEP here. There are distinct fields for author and author email, so are we sure that all RFC 822 formats should be accepted? Would this break the various Metadata file parsers?
Same question for multiple authors.
> I am not sure that the definition of the format matches the data model of the Metadata PEP here. There are distinct fields for author and author email, so are we sure that all RFC 822 formats should be accepted?
@merwok If I follow you correctly, you're pointing out that a RFC 822 style email may contain the author's name, which seems redundant when there is an `Author` field as well. I don't think this is a problem.
> Would this break the various Metadata file parsers?
I don't think this would break anything in Warehouse. The `pkginfo` project already interprets these fields via the `email` stdlib module, so I don't think it would break anything there either. To my knowledge, there isn't anything that depends on these fields being a single valid email address, because this restriction was a relatively new addition and there are a fair amount of packages that don't even have valid RFC 822 style values.
To be clear, this should be considered a regression: there are packages on PyPI already that are using this format for these fields, which were uploaded to pypi-legacy, and would fail if they were uploaded to Warehouse today.
> @pradyunsg hasn't submitted a PR for this issue (yet!)
Yeah... I've been swamped by some other work, so, I've not been able to get to this.
Hmm, so my thinking when I added the new validation was that currently people would be just complete garbage in that field sometimes. I think validating data here is still the right thing to do (because having nonsense values is bad, even if previous releases were able to upload with that). I don't think that it's a bad idea to allow the RFC 822 email addresses here though, though it raises the question of how we should display it. Right now we generate a link that looks like``<a href="mailto:THEEMAILADDRESS">THEAUTHORNAME</a>``, but what do we do if we have ``Author-Name``, and an email address like ``Foo <[email protected]>``, do we just extract the email address? Change the display?
@dstufft I think that anything that is a valid RFC 822 value _should_ be a valid value for a a `mailto` link, e.g. <a href="mailto: Foo <[email protected]>">a link like this</a> would work just fine.
Heh, welp. I guess I forgot that worked. | 2018-02-08T23:01:12Z | [] | [] |
pypi/warehouse | 2,907 | pypi__warehouse-2907 | [
"2828"
] | bf0495b105d1b0738aa24a2a1f9b19811d68d68e | diff --git a/warehouse/manage/views.py b/warehouse/manage/views.py
--- a/warehouse/manage/views.py
+++ b/warehouse/manage/views.py
@@ -71,7 +71,15 @@ def save_profile(self):
effective_principals=Authenticated,
)
def manage_projects(request):
- return {}
+
+ def _key(project):
+ if project.releases:
+ return project.releases[0].created
+ return project.created
+
+ return {
+ 'projects': sorted(request.user.projects, key=_key, reverse=True)
+ }
@view_config(
diff --git a/warehouse/packaging/models.py b/warehouse/packaging/models.py
--- a/warehouse/packaging/models.py
+++ b/warehouse/packaging/models.py
@@ -175,6 +175,16 @@ def documentation_url(self):
return request.route_url("legacy.docs", project=self.name)
+ @property
+ def owners(self):
+ return (
+ orm.object_session(self)
+ .query(User)
+ .join(Role.user)
+ .filter(Role.project == self, Role.role_name == 'Owner')
+ .all()
+ )
+
class DependencyKind(enum.IntEnum):
| diff --git a/tests/unit/manage/test_views.py b/tests/unit/manage/test_views.py
--- a/tests/unit/manage/test_views.py
+++ b/tests/unit/manage/test_views.py
@@ -111,9 +111,33 @@ def test_save_profile_validation_fails(self, monkeypatch):
class TestManageProjects:
def test_manage_projects(self):
- request = pretend.stub()
+ project_with_older_release = pretend.stub(
+ releases=[pretend.stub(created=0)]
+ )
+ project_with_newer_release = pretend.stub(
+ releases=[pretend.stub(created=2)]
+ )
+ older_project_with_no_releases = pretend.stub(releases=[], created=1)
+ newer_project_with_no_releases = pretend.stub(releases=[], created=3)
+ request = pretend.stub(
+ user=pretend.stub(
+ projects=[
+ project_with_older_release,
+ project_with_newer_release,
+ newer_project_with_no_releases,
+ older_project_with_no_releases,
+ ],
+ ),
+ )
- assert views.manage_projects(request) == {}
+ assert views.manage_projects(request) == {
+ 'projects': [
+ newer_project_with_no_releases,
+ project_with_newer_release,
+ older_project_with_no_releases,
+ project_with_older_release,
+ ],
+ }
class TestManageProjectSettings:
diff --git a/tests/unit/packaging/test_models.py b/tests/unit/packaging/test_models.py
--- a/tests/unit/packaging/test_models.py
+++ b/tests/unit/packaging/test_models.py
@@ -116,6 +116,15 @@ def test_acl(self, db_session):
(Allow, str(maintainer2.user.id), ["upload"]),
]
+ def test_owners(self, db_session):
+ project = DBProjectFactory.create()
+ owner1 = DBRoleFactory.create(project=project)
+ owner2 = DBRoleFactory.create(project=project)
+ DBRoleFactory.create(project=project, role_name="Maintainer")
+ DBRoleFactory.create(project=project, role_name="Maintainer")
+
+ assert set(project.owners) == set([owner1.user, owner2.user])
+
class TestRelease:
| Disable "view project" links when project has no releases
Currently in the logged-in UI, there are a few places where there are "View" and "View Project" buttons for a maintainer's project, which lead to the project page (e.g. `https://pypi.org/project/<project_name>/`)
However if the project has no releases, the project page will be 404. In this case, we should disable these buttons (via the `disabled` attribute) and add an alt text saying something like "This project has no releases".
| 2018-02-09T22:28:59Z | [] | [] |
|
pypi/warehouse | 2,911 | pypi__warehouse-2911 | [
"2871"
] | b35fbb69d02d698a578720230696bdcdd93729f6 | diff --git a/warehouse/manage/views.py b/warehouse/manage/views.py
--- a/warehouse/manage/views.py
+++ b/warehouse/manage/views.py
@@ -462,3 +462,22 @@ def delete_project_role(project, request):
return HTTPSeeOther(
request.route_path('manage.project.roles', project_name=project.name)
)
+
+
+@view_config(
+ route_name="manage.project.history",
+ renderer="manage/history.html",
+ uses_session=True,
+ permission="manage",
+)
+def manage_project_history(project, request):
+ journals = (
+ request.db.query(JournalEntry)
+ .filter(JournalEntry.name == project.name)
+ .order_by(JournalEntry.submitted_date.desc())
+ .all()
+ )
+ return {
+ 'project': project,
+ 'journals': journals,
+ }
diff --git a/warehouse/routes.py b/warehouse/routes.py
--- a/warehouse/routes.py
+++ b/warehouse/routes.py
@@ -162,6 +162,13 @@ def includeme(config):
traverse="/{project_name}",
domain=warehouse,
)
+ config.add_route(
+ "manage.project.history",
+ "/manage/project/{project_name}/history/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{project_name}",
+ domain=warehouse,
+ )
# Packaging
config.add_route(
| diff --git a/tests/unit/manage/test_views.py b/tests/unit/manage/test_views.py
--- a/tests/unit/manage/test_views.py
+++ b/tests/unit/manage/test_views.py
@@ -10,6 +10,7 @@
# See the License for the specific language governing permissions and
# limitations under the License.
+import datetime
import uuid
import pretend
@@ -22,7 +23,9 @@
from warehouse.accounts.interfaces import IUserService
from warehouse.packaging.models import JournalEntry, Project, Role
-from ...common.db.packaging import ProjectFactory, RoleFactory, UserFactory
+from ...common.db.packaging import (
+ JournalEntryFactory, ProjectFactory, RoleFactory, UserFactory,
+)
class TestManageProfile:
@@ -974,3 +977,22 @@ def test_delete_own_owner_role(self, db_request):
]
assert isinstance(result, HTTPSeeOther)
assert result.headers["Location"] == "/the-redirect"
+
+
+class TestManageProjectHistory:
+
+ def test_get(self, db_request):
+ project = ProjectFactory.create()
+ older_journal = JournalEntryFactory.create(
+ name=project.name,
+ submitted_date=datetime.datetime(2017, 2, 5, 17, 18, 18, 462634),
+ )
+ newer_journal = JournalEntryFactory.create(
+ name=project.name,
+ submitted_date=datetime.datetime(2018, 2, 5, 17, 18, 18, 462634),
+ )
+
+ assert views.manage_project_history(project, db_request) == {
+ 'project': project,
+ 'journals': [newer_journal, older_journal],
+ }
diff --git a/tests/unit/test_routes.py b/tests/unit/test_routes.py
--- a/tests/unit/test_routes.py
+++ b/tests/unit/test_routes.py
@@ -196,6 +196,13 @@ def add_policy(name, filename):
traverse="/{project_name}",
domain=warehouse,
),
+ pretend.call(
+ "manage.project.history",
+ "/manage/project/{project_name}/history/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{project_name}",
+ domain=warehouse,
+ ),
pretend.call(
"packaging.project",
"/project/{name}/",
| Display project/release journal
Sub ticket of #2734
We should show the maintainer some history of their project/release. The current UI looks like this:
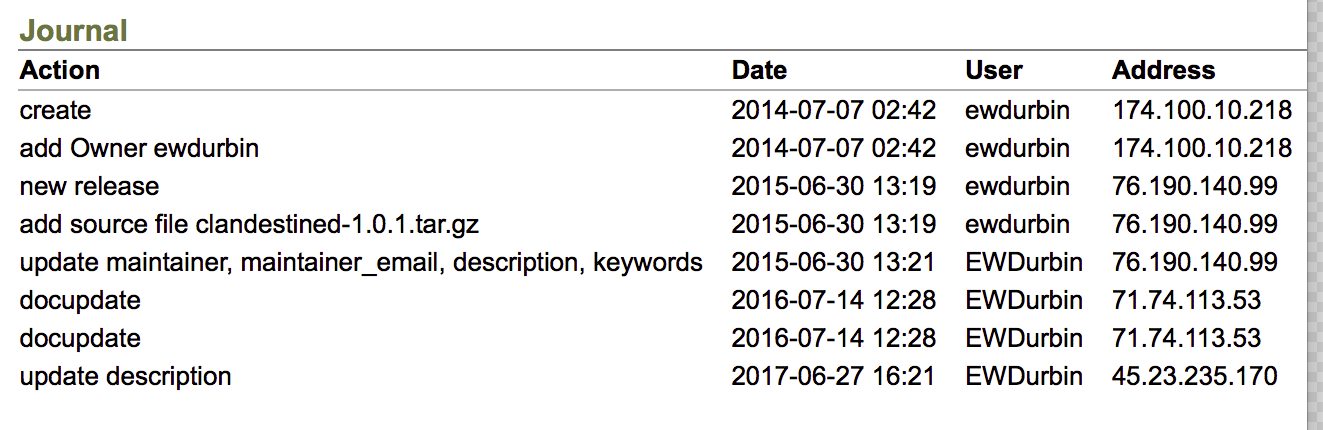
Questions:
- Should this be on a project level?
- Should this be on a release level?
- Should it be on *both*?
- What milestone should it be in?
On the last question, it's probably not a heap of work for me to get it into the maintainer MVP - so long as the data is ready to go :)
ping @di @ewdurbin @brainwane @dstufft
| from @di (https://github.com/pypa/warehouse/issues/2807#issuecomment-362085418)
> Hmm, looks like I'm wrong -- legacy PyPI actually does show a mix of project-level journal entries and release-level journal entries on each release page.
>
> This seems like it would be somewhat confusing to users. IMO it'd be better to have all the journal entries in one place, but perhaps I'm missing something obvious and @ewdurbin can chime in and explain why it's like that on legacy.
I put this in the Maintainer MVP milestone, since it already exists (in some form) on legacy. | 2018-02-10T11:20:52Z | [] | [] |
pypi/warehouse | 2,922 | pypi__warehouse-2922 | [
"2887"
] | 1f1c3869b369662e084d1bfa0a2a440270450448 | diff --git a/warehouse/accounts/forms.py b/warehouse/accounts/forms.py
--- a/warehouse/accounts/forms.py
+++ b/warehouse/accounts/forms.py
@@ -185,7 +185,10 @@ def __init__(self, *args, user_service, **kwargs):
self.user_service = user_service
-class RequestPasswordResetForm(UsernameMixin, forms.Form):
+class RequestPasswordResetForm(forms.Form):
+ username_or_email = wtforms.StringField(
+ validators=[wtforms.validators.DataRequired()]
+ )
def __init__(self, *args, user_service, **kwargs):
super().__init__(*args, **kwargs)
diff --git a/warehouse/accounts/interfaces.py b/warehouse/accounts/interfaces.py
--- a/warehouse/accounts/interfaces.py
+++ b/warehouse/accounts/interfaces.py
@@ -47,6 +47,12 @@ def get_user_by_username(username):
if there is no user with that username.
"""
+ def get_user_by_email(email):
+ """
+ Return the user object corresponding with the given email, or None
+ if there is no user with that email.
+ """
+
def find_userid(username):
"""
Find the unique user identifier for the given username or None if there
diff --git a/warehouse/accounts/services.py b/warehouse/accounts/services.py
--- a/warehouse/accounts/services.py
+++ b/warehouse/accounts/services.py
@@ -76,6 +76,11 @@ def get_user_by_username(self, username):
user_id = self.find_userid(username)
return None if user_id is None else self.get_user(user_id)
+ @functools.lru_cache()
+ def get_user_by_email(self, email):
+ user_id = self.find_userid_by_email(email)
+ return None if user_id is None else self.get_user(user_id)
+
@functools.lru_cache()
def find_userid(self, username):
try:
diff --git a/warehouse/accounts/views.py b/warehouse/accounts/views.py
--- a/warehouse/accounts/views.py
+++ b/warehouse/accounts/views.py
@@ -268,9 +268,15 @@ def request_password_reset(request, _form_class=RequestPasswordResetForm):
form = _form_class(request.POST, user_service=user_service)
if request.method == "POST" and form.validate():
- user = user_service.get_user_by_username(form.username.data)
- fields = send_password_reset_email(request, user)
- return {'n_hours': fields['n_hours']}
+ user = user_service.get_user_by_username(form.username_or_email.data)
+ if user is None:
+ user = user_service.get_user_by_email(form.username_or_email.data)
+ if user:
+ send_password_reset_email(request, user)
+
+ token_service = request.find_service(ITokenService, name='password')
+ n_hours = token_service.max_age // 60 // 60
+ return {"n_hours": n_hours}
return {"form": form}
| diff --git a/tests/unit/accounts/test_forms.py b/tests/unit/accounts/test_forms.py
--- a/tests/unit/accounts/test_forms.py
+++ b/tests/unit/accounts/test_forms.py
@@ -365,29 +365,6 @@ def test_no_password_field(self):
form = forms.RequestPasswordResetForm(user_service=user_service)
assert 'password' not in form._fields
- def test_validate_username_with_no_user(self):
- user_service = pretend.stub(
- find_userid=pretend.call_recorder(lambda userid: None),
- )
- form = forms.RequestPasswordResetForm(user_service=user_service)
- field = pretend.stub(data="my_username")
-
- with pytest.raises(wtforms.validators.ValidationError):
- form.validate_username(field)
-
- assert user_service.find_userid.calls == [pretend.call("my_username")]
-
- def test_validate_username_with_user(self):
- user_service = pretend.stub(
- find_userid=pretend.call_recorder(lambda userid: 1),
- )
- form = forms.RequestPasswordResetForm(user_service=user_service)
- field = pretend.stub(data="my_username")
-
- form.validate_username(field)
-
- assert user_service.find_userid.calls == [pretend.call("my_username")]
-
class TestResetPasswordForm:
diff --git a/tests/unit/accounts/test_services.py b/tests/unit/accounts/test_services.py
--- a/tests/unit/accounts/test_services.py
+++ b/tests/unit/accounts/test_services.py
@@ -213,6 +213,20 @@ def test_get_user_by_username_failure(self, user_service):
assert found_user is None
+ def test_get_user_by_email(self, user_service):
+ user = UserFactory.create()
+ EmailFactory.create(user=user, primary=True, verified=False)
+ found_user = user_service.get_user_by_email(user.emails[0].email)
+ user_service.db.flush()
+
+ assert user.id == found_user.id
+
+ def test_get_user_by_email_failure(self, user_service):
+ found_user = user_service.get_user_by_email("[email protected]")
+ user_service.db.flush()
+
+ assert found_user is None
+
class TestTokenService:
diff --git a/tests/unit/accounts/test_views.py b/tests/unit/accounts/test_views.py
--- a/tests/unit/accounts/test_views.py
+++ b/tests/unit/accounts/test_views.py
@@ -390,7 +390,7 @@ def test_get(self, pyramid_request, user_service):
form_inst = pretend.stub()
form_class = pretend.call_recorder(lambda *args, **kwargs: form_inst)
pyramid_request.find_service = pretend.call_recorder(
- lambda *args, **kwargs: user_service
+ lambda *a, **kw: user_service,
)
pyramid_request.POST = pretend.stub()
result = views.request_password_reset(
@@ -416,14 +416,17 @@ def test_request_password_reset(
lambda a: stub_user
)
pyramid_request.find_service = pretend.call_recorder(
- lambda *a, **kw: user_service,
+ lambda interface, **kw: {
+ IUserService: user_service,
+ ITokenService: token_service,
+ }[interface],
)
form_obj = pretend.stub(
- username=pretend.stub(data=stub_user.username),
+ username_or_email=pretend.stub(data=stub_user.username),
validate=pretend.call_recorder(lambda: True),
)
form_class = pretend.call_recorder(lambda d, user_service: form_obj)
- n_hours = pretend.stub()
+ n_hours = token_service.max_age // 60 // 60
send_password_reset_email = pretend.call_recorder(
lambda *args, **kwargs: {'n_hours': n_hours},
)
@@ -441,6 +444,7 @@ def test_request_password_reset(
]
assert pyramid_request.find_service.calls == [
pretend.call(IUserService, context=None),
+ pretend.call(ITokenService, name='password'),
]
assert form_obj.validate.calls == [
pretend.call(),
@@ -452,6 +456,118 @@ def test_request_password_reset(
pretend.call(pyramid_request, stub_user),
]
+ def test_request_password_reset_with_email(
+ self, monkeypatch, pyramid_request, pyramid_config, user_service,
+ token_service):
+
+ stub_user = pretend.stub(email=pretend.stub())
+ pyramid_request.method = "POST"
+ token_service.dumps = pretend.call_recorder(lambda a: "TOK")
+ user_service.get_user_by_username = pretend.call_recorder(
+ lambda a: None
+ )
+ user_service.get_user_by_email = pretend.call_recorder(
+ lambda a: stub_user
+ )
+ pyramid_request.find_service = pretend.call_recorder(
+ lambda interface, **kw: {
+ IUserService: user_service,
+ ITokenService: token_service,
+ }[interface],
+ )
+ form_obj = pretend.stub(
+ username_or_email=pretend.stub(data=stub_user.email),
+ validate=pretend.call_recorder(lambda: True),
+ )
+ form_class = pretend.call_recorder(lambda d, user_service: form_obj)
+ n_hours = token_service.max_age // 60 // 60
+ send_password_reset_email = pretend.call_recorder(
+ lambda *args, **kwargs: {'n_hours': n_hours},
+ )
+ monkeypatch.setattr(
+ views, 'send_password_reset_email', send_password_reset_email
+ )
+
+ result = views.request_password_reset(
+ pyramid_request, _form_class=form_class
+ )
+
+ assert result == {'n_hours': n_hours}
+ assert user_service.get_user_by_username.calls == [
+ pretend.call(stub_user.email),
+ ]
+ assert user_service.get_user_by_email.calls == [
+ pretend.call(stub_user.email),
+ ]
+ assert pyramid_request.find_service.calls == [
+ pretend.call(IUserService, context=None),
+ pretend.call(ITokenService, name='password'),
+ ]
+ assert form_obj.validate.calls == [
+ pretend.call(),
+ ]
+ assert form_class.calls == [
+ pretend.call(pyramid_request.POST, user_service=user_service),
+ ]
+ assert send_password_reset_email.calls == [
+ pretend.call(pyramid_request, stub_user),
+ ]
+
+ def test_request_password_reset_with_wrong_credentials(
+ self, monkeypatch, pyramid_request, pyramid_config, user_service,
+ token_service):
+
+ stub_user = pretend.stub(username=pretend.stub())
+ pyramid_request.method = "POST"
+ token_service.dumps = pretend.call_recorder(lambda a: "TOK")
+ user_service.get_user_by_username = pretend.call_recorder(
+ lambda a: None
+ )
+ user_service.get_user_by_email = pretend.call_recorder(
+ lambda a: None
+ )
+ pyramid_request.find_service = pretend.call_recorder(
+ lambda interface, **kw: {
+ IUserService: user_service,
+ ITokenService: token_service,
+ }[interface],
+ )
+ form_obj = pretend.stub(
+ username_or_email=pretend.stub(data=stub_user.username),
+ validate=pretend.call_recorder(lambda: True),
+ )
+ form_class = pretend.call_recorder(lambda d, user_service: form_obj)
+ n_hours = token_service.max_age // 60 // 60
+ send_password_reset_email = pretend.call_recorder(
+ lambda *args, **kwargs: {'n_hours': n_hours},
+ )
+ monkeypatch.setattr(
+ views, 'send_password_reset_email', send_password_reset_email
+ )
+
+ result = views.request_password_reset(
+ pyramid_request, _form_class=form_class
+ )
+
+ assert result == {'n_hours': n_hours}
+ assert user_service.get_user_by_username.calls == [
+ pretend.call(stub_user.username),
+ ]
+ assert user_service.get_user_by_email.calls == [
+ pretend.call(stub_user.username),
+ ]
+ assert pyramid_request.find_service.calls == [
+ pretend.call(IUserService, context=None),
+ pretend.call(ITokenService, name='password'),
+ ]
+ assert form_obj.validate.calls == [
+ pretend.call(),
+ ]
+ assert form_class.calls == [
+ pretend.call(pyramid_request.POST, user_service=user_service),
+ ]
+ assert send_password_reset_email.calls == []
+
class TestResetPassword:
| Account recovery by Email
If you try to do "Request Password Reset" you'll face a problem. The form requires your login that most of the users don't know or forget. It'll be very useful to do account recovery by Email, isn't it?
| 2018-02-12T16:37:30Z | [] | [] |
|
pypi/warehouse | 2,942 | pypi__warehouse-2942 | [
"2940"
] | 2f6c867a89714f029b64e4098bf1c320c2fb1edf | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -52,10 +52,19 @@
PATH_HASHER = "blake2_256"
+
+def namespace_stdlib_list(module_list):
+ for module_name in module_list:
+ parts = module_name.split('.')
+ for i, part in enumerate(parts):
+ yield '.'.join(parts[:i + 1])
+
+
STDLIB_PROHIBITTED = {
packaging.utils.canonicalize_name(s.rstrip('-_.').lstrip('-_.'))
- for s in chain.from_iterable(stdlib_list.stdlib_list(version)
- for version in stdlib_list.short_versions)
+ for s in chain.from_iterable(
+ namespace_stdlib_list(stdlib_list.stdlib_list(version))
+ for version in stdlib_list.short_versions)
}
# Wheel platform checking
| diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -882,6 +882,11 @@ def test_fails_with_invalid_names(self, pyramid_config, db_request, name):
"main", "future", "al", "uU", "test",
"encodings.utf_8_sig",
"distutils.command.build_clib",
+ "xmlrpc", "xmlrpc.server",
+ "xml.etree", "xml.etree.ElementTree",
+ "xml.parsers", "xml.parsers.expat",
+ "xml.parsers.expat.errors",
+ "encodings.idna", "encodings",
"CGIHTTPServer", "cgihttpserver"])
def test_fails_with_stdlib_names(self, pyramid_config, db_request, name):
pyramid_config.testing_securitypolicy(userid=1)
| Blocking of stdlib names has holes?
Hi!
Pull request #2409 introduced a blacklist of stdlib names to counter to protect against [pytosquatting](https://www.pytosquatting.org/), aiming to plug #2151.
I found a hole with it. While names like `xmlrpc.client` and `encodings.idna` are blocked because they appear in some `stdlib-list` list, some parent packages like `encodings` are not in their list and do not get blocked by warehouse as a consequence. I was able to register these four packages:
* [encodings](https://pypi.org/project/encodings/)
* [xml.etree](https://pypi.org/project/xml.etree/)
* [xml.parsers](https://pypi.org/project/xml.parsers/)
* [xmlrpc](https://pypi.org/project/xmlrpc/)
According to [pytosquatting.org](https://www.pytosquatting.org/), package `encodings` had about 20 hits per day in the past, not sure how much hits the others would get. Personally, I would have considered at least `encodings` and `xmlrpc` worth blocking.
Should `stdlib-list` be completed or rather parent package names auto-included at warehouse level for a generic fix? What do you think?
PS: I'm happy to delete these four packages from PyPI once this hole is plugged. I would ask to keep them up until then, please feel free to verify their whitehat nature. Thank you!
Best, Sebastian
CC: @benjaoming @hannob
| @hartwork thanks for the report. interesting work around :)
i'm going to get a fix in at the warehouse level, then pull down the registered packages.
in the future, PyPI does have a security policy published at https://pypi.org/security/ and https://pypi.python.org/security for issues relating to the security of our service or our users. | 2018-02-14T20:14:48Z | [] | [] |
pypi/warehouse | 2,948 | pypi__warehouse-2948 | [
"1886"
] | d980bcff01366a2cfa9afcab6fcad8f55a588d2d | diff --git a/warehouse/legacy/api/xmlrpc.py b/warehouse/legacy/api/xmlrpc.py
--- a/warehouse/legacy/api/xmlrpc.py
+++ b/warehouse/legacy/api/xmlrpc.py
@@ -83,9 +83,9 @@ def search(request, spec, operator="and"):
q = None
for item in value:
if q is None:
- q = Q("match", **{field: item})
+ q = Q("term", **{field: item})
else:
- q |= Q("match", **{field: item})
+ q |= Q("term", **{field: item})
queries.append(q)
if operator == "and":
| diff --git a/tests/unit/legacy/api/test_xmlrpc.py b/tests/unit/legacy/api/test_xmlrpc.py
--- a/tests/unit/legacy/api/test_xmlrpc.py
+++ b/tests/unit/legacy/api/test_xmlrpc.py
@@ -57,12 +57,12 @@ def __getitem__(self, name):
def execute(self):
assert self.type == "bool"
assert [q.to_dict() for q in self.must] == [
- {"match": {"name": "foo"}},
+ {"term": {"name": "foo"}},
{
"bool": {
"should": [
- {"match": {"summary": "one"}},
- {"match": {"summary": "two"}},
+ {"term": {"summary": "one"}},
+ {"term": {"summary": "two"}},
],
},
},
@@ -94,6 +94,53 @@ def execute(self):
{"name": "foo-bar", "summary": "other summary", "version": "1.0"},
]
+ def test_default_search_operator_with_spaces_in_values(self):
+ class FakeQuery:
+ def __init__(self, type, must):
+ self.type = type
+ self.must = must
+
+ def __getitem__(self, name):
+ self.offset = name.start
+ self.limit = name.stop
+ self.step = name.step
+ return self
+
+ def execute(self):
+ assert self.type == "bool"
+ assert [q.to_dict() for q in self.must] == [
+ {'bool': {'should': [
+ {'term': {'summary': 'fix code'}},
+ {'term': {'summary': 'like this'}}
+ ]}}
+ ]
+ assert self.offset is None
+ assert self.limit == 1000
+ assert self.step is None
+ return [
+ pretend.stub(
+ name="foo",
+ summary="fix code",
+ version=["1.0"],
+ ),
+ pretend.stub(
+ name="foo-bar",
+ summary="like this",
+ version=["2.0", "1.0"],
+ ),
+ ]
+
+ request = pretend.stub(es=pretend.stub(query=FakeQuery))
+ results = xmlrpc.search(
+ request,
+ {"summary": ["fix code", "like this"]},
+ )
+ assert results == [
+ {"name": "foo", "summary": "fix code", "version": "1.0"},
+ {"name": "foo-bar", "summary": "like this", "version": "2.0"},
+ {"name": "foo-bar", "summary": "like this", "version": "1.0"},
+ ]
+
def test_searches_with_and(self):
class FakeQuery:
def __init__(self, type, must):
@@ -109,12 +156,12 @@ def __getitem__(self, name):
def execute(self):
assert self.type == "bool"
assert [q.to_dict() for q in self.must] == [
- {"match": {"name": "foo"}},
+ {"term": {"name": "foo"}},
{
"bool": {
"should": [
- {"match": {"summary": "one"}},
- {"match": {"summary": "two"}},
+ {"term": {"summary": "one"}},
+ {"term": {"summary": "two"}},
],
},
},
@@ -162,12 +209,12 @@ def __getitem__(self, name):
def execute(self):
assert self.type == "bool"
assert [q.to_dict() for q in self.should] == [
- {"match": {"name": "foo"}},
+ {"term": {"name": "foo"}},
{
"bool": {
"should": [
- {"match": {"summary": "one"}},
- {"match": {"summary": "two"}},
+ {"term": {"summary": "one"}},
+ {"term": {"summary": "two"}},
],
},
},
| Search method in XML-RPC API treats {"summary": "fix code"} as {"summary": ["fix", "code"]}
As stated in the title, the `search` method of [PyPI/Warehouse's XML-RPC API](https://warehouse.readthedocs.io/api-reference/xml-rpc/) splits the values of its `spec` argument on whitespace, resulting in the method returning (for the example in the title) packages with either "fix" or "code" in their summary, not just those with both "fix" and "code" (let alone just those that contain the exact string "fix code"). This happens even if the `spec` value is passed as a one-element list (e.g., `{"summary": ["fix code"]}`), and while I haven't tested every field, it is not limited to just "summary".
On the off chance that this is the intended behavior, the documentation should at least be updated.
| We discussed this in [today's Warehouse bug triage meeting](https://wiki.python.org/psf/PackagingWG/2018-01-29-Warehouse) and decided this is probably a small fix, we need to compare it to behavior on legacy PyPI, and we'll address it in the End User MVP milestone (probably in March). Thanks, @jwodder!
@waseem18 I saw you're working on this? Thanks! Hope you'll join us [in Freenode IRC in `#pypa-dev`](https://webchat.freenode.net/?channels=%23pypa-dev) if you have any questions.
@brainwane Yeah I've started working on this an will put a WIP PR soon.
**Cause of this bug** :
In `xmlrpc.py` line number [86](https://github.com/pypa/warehouse/blob/747b054c798b25dd43d86a75a5120a3245e153ac/warehouse/legacy/api/xmlrpc.py#L86) and 88 we're using `match query` to query elasticsearch for the given value.
In `match query` what happens is when the value of the given field has space(s) it tokenizes the given value thereby searching for each of the split tokens.
`"query": {
"match" : {
"summary" : "fix code"
}
}`
Above is a `match query` with space in the value of the field `summary`. So it tokenizes the value to `["fix", "code"]` and searches for `fix` or `code`.
**Resolution** :
Use [Term query](https://www.elastic.co/guide/en/elasticsearch/reference/current/query-dsl-term-query.html#query-dsl-term-query) when the value of the given field has space(s) and `match query` when the value doesn't have any space(s).
#2850 is similar to this issue. Using Term query / `match_phrase` when the entered value has double quotes.
@di @brainwane Will be happy to get your feedback on this.
@waseem18 Using the `term` query makes sense, especially considering the API allows for multiple strings to be provided.
Any reason why we wouldn't just use it for queries without whitespace as well? Seems like it wouldn't make much difference if the user is just searching for a single word.
For #2850, it seems like we might actually want to do [Phrase Matching](https://www.elastic.co/guide/en/elasticsearch/guide/current/phrase-matching.html) instead, but let's just focus on this issue for now.
Good catch @di
Using `term` query for values without space wouldn't make much difference. I'll go with it :+1: | 2018-02-15T17:58:19Z | [] | [] |
pypi/warehouse | 2,955 | pypi__warehouse-2955 | [
"2788"
] | 1a3f5d9d6a2bf5677f8dca00e6d1b9cf49cfdb89 | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -811,53 +811,69 @@ def file_upload(request):
(Release.version == form.version.data)).one()
)
except NoResultFound:
- release = Release(
- project=project,
- _classifiers=[
- c for c in all_classifiers
- if c.classifier in form.classifiers.data
- ],
- _pypi_hidden=False,
- dependencies=list(_construct_dependencies(
- form,
- {
- "requires": DependencyKind.requires,
- "provides": DependencyKind.provides,
- "obsoletes": DependencyKind.obsoletes,
- "requires_dist": DependencyKind.requires_dist,
- "provides_dist": DependencyKind.provides_dist,
- "obsoletes_dist": DependencyKind.obsoletes_dist,
- "requires_external": DependencyKind.requires_external,
- "project_urls": DependencyKind.project_url,
- }
- )),
- **{
- k: getattr(form, k).data
- for k in {
- # This is a list of all the fields in the form that we
- # should pull off and insert into our new release.
- "version",
- "summary", "description", "license",
- "author", "author_email", "maintainer", "maintainer_email",
- "keywords", "platform",
- "home_page", "download_url",
- "requires_python",
- }
- }
- )
- request.db.add(release)
- # TODO: This should be handled by some sort of database trigger or a
- # SQLAlchemy hook or the like instead of doing it inline in this
- # view.
- request.db.add(
- JournalEntry(
- name=release.project.name,
- version=release.version,
- action="new release",
- submitted_by=request.user,
- submitted_from=request.remote_addr,
- ),
+ # We didn't find a release with the exact version string, try and see
+ # if one exists with an equivalent parsed version
+ releases = (
+ request.db.query(Release)
+ .filter(Release.project == project)
+ .all()
)
+ versions = {
+ packaging.version.parse(release.version): release
+ for release in releases
+ }
+
+ try:
+ release = versions[packaging.version.parse(form.version.data)]
+ except KeyError:
+ release = Release(
+ project=project,
+ _classifiers=[
+ c for c in all_classifiers
+ if c.classifier in form.classifiers.data
+ ],
+ _pypi_hidden=False,
+ dependencies=list(_construct_dependencies(
+ form,
+ {
+ "requires": DependencyKind.requires,
+ "provides": DependencyKind.provides,
+ "obsoletes": DependencyKind.obsoletes,
+ "requires_dist": DependencyKind.requires_dist,
+ "provides_dist": DependencyKind.provides_dist,
+ "obsoletes_dist": DependencyKind.obsoletes_dist,
+ "requires_external": DependencyKind.requires_external,
+ "project_urls": DependencyKind.project_url,
+ }
+ )),
+ **{
+ k: getattr(form, k).data
+ for k in {
+ # This is a list of all the fields in the form that we
+ # should pull off and insert into our new release.
+ "version",
+ "summary", "description", "license",
+ "author", "author_email",
+ "maintainer", "maintainer_email",
+ "keywords", "platform",
+ "home_page", "download_url",
+ "requires_python",
+ }
+ }
+ )
+ request.db.add(release)
+ # TODO: This should be handled by some sort of database trigger or
+ # a SQLAlchemy hook or the like instead of doing it inline in
+ # this view.
+ request.db.add(
+ JournalEntry(
+ name=release.project.name,
+ version=release.version,
+ action="new release",
+ submitted_by=request.user,
+ submitted_from=request.remote_addr,
+ ),
+ )
# TODO: We need a better solution to this than to just do it inline inside
# this method. Ideally the version field would just be sortable, but
| diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -2253,6 +2253,52 @@ def test_upload_succeeds_creates_release(self, pyramid_config, db_request):
),
]
+ def test_equivalent_version_one_release(self, pyramid_config, db_request):
+ '''
+ Test that if a release with a version like '1.0' exists, that a future
+ upload with an equivalent version like '1.0.0' will not make a second
+ release
+ '''
+ pyramid_config.testing_securitypolicy(userid=1)
+
+ user = UserFactory.create()
+ project = ProjectFactory.create()
+ release = ReleaseFactory.create(project=project, version="1.0")
+ RoleFactory.create(user=user, project=project)
+
+ db_request.user = user
+ db_request.remote_addr = "10.10.10.20"
+ db_request.POST = MultiDict({
+ "metadata_version": "1.2",
+ "name": project.name,
+ "version": "1.0.0",
+ "summary": "This is my summary!",
+ "filetype": "sdist",
+ "md5_digest": "335c476dc930b959dda9ec82bd65ef19",
+ "content": pretend.stub(
+ filename="{}-{}.tar.gz".format(project.name, "1.0.0"),
+ file=io.BytesIO(b"A fake file."),
+ type="application/tar",
+ ),
+ })
+
+ storage_service = pretend.stub(store=lambda path, filepath, meta: None)
+ db_request.find_service = lambda svc: storage_service
+
+ resp = legacy.file_upload(db_request)
+
+ assert resp.status_code == 200
+
+ # Ensure that a Release object has been created.
+ releases = (
+ db_request.db.query(Release)
+ .filter(Release.project == project)
+ .all()
+ )
+
+ # Asset that only one release has been created
+ assert releases == [release]
+
def test_upload_succeeds_creates_project(self, pyramid_config, db_request):
pyramid_config.testing_securitypolicy(userid=1)
| Inconsistent handling of packages with equivalent version numbers (e.g. v4.1 & v4.1.0)
## Situation
Yesterday I uploaded a new release (**v4.1**) of 'theonionbox' to `https://upload.pypi.org/legacy/`.
Short after the upload I discovered that I made a mistake in the packaging process. With the intension to fix this 'silently' = without (relevant) change of the version number, I created a **v4.1.0** package and pushed it to PyPI. PyPI accepted this without complaint.
## Finding
Today, the comparison of the legacy system [pypi.python.org](https://pypi.python.org/) vs the _warehouse_ based [new one](https://pypi.org/) reveals what looks like a difference in the version number sorting algorithm:
[pypi.python.org](https://pypi.python.org/pypi/theonionbox) offers v4.1.0 as the latest version...
<p align='center'>
<img width="80%" alt="4 1 0" src="https://user-images.githubusercontent.com/16342003/34959213-c6736858-fa35-11e7-8f05-11924201219a.png">
</p>
... whereas [pypi.org](https://pypi.org/project/theonionbox/) announces v4.1:
<p align='center'>
<img width="80%" alt="4 1" src="https://user-images.githubusercontent.com/16342003/34959257-02eec3a4-fa36-11e7-81f3-d71f56c5b750.png">
</p>
## Some further remarks
- The [Python source code](https://svn.python.org/projects/stackless/trunk/Lib/distutils/version.py) explains that v4.1 and v4.1.0 are equivalent:
<p align='center'>
<img width="80%" alt="strict" src="https://user-images.githubusercontent.com/16342003/34959520-298f806a-fa37-11e7-96a6-5c48bccae081.png">
</p>
- Performing a `pip install theonionbox` collects v4.1.
- Performing an explicit `pip install theonionbox==4.1.0` collects v4.1 as well.
- The sequencing in the [release history](https://pypi.org/project/theonionbox/#history) of pypi.org displays v4.1.0 prior to v4.1 - which is technically wrong with regard to the upload time:
<p align='center'>
<img width="80%" alt="history" src="https://user-images.githubusercontent.com/16342003/34959868-aaec8292-fa38-11e7-98d1-f3cae7d93fdb.png">
</p>
- Explicitly selecting v4.1.0, [pypi.org](https://pypi.org/project/theonionbox/4.1.0/) as well states that this is the 'Latest Version':
<p align='center'>
<img width="80%" alt="latest" src="https://user-images.githubusercontent.com/16342003/34960655-04808aee-fa3c-11e7-91d4-4eadcd63b649.png">
</p>
- After a click on this 'Latest Version' button ... pypi.org crashed. :anguished:
## Summary
- pypi.python.org and pypi.org are not consistent in the way they handle this special case of version numbering.
- One could argue that pypi.python.org is 'more correct' as it respects the sequencing of the file upload.
## Proposal
- Option A: Change _warehouse_ to follow the legacy algorithm.
- Option B: As the Python source explicitly states, that v4.1 and v4.1.0 are equivalent, _warehouse_ should treat them as equivalent and reject uploads of that kind.
My preference is **Option B**.
| @ralphwetzel Thanks for your detailed and thorough bug report, and sorry for the slow response!
I spoke with @di about this and we agree with **Option B**:
~~~
>>> from packaging.version import parse
>>> parse('4.1') == parse('4.1.0')
True
~~~
You mentioned that pypi.org crashed when you tried to select ‘Latest Version’, and that button ought to go to the page you were already on. Do you remember any details about the crash? Was it an error 500?
Thanks and sorry again for the wait.
For future developers who want to fix this: We get the release for a file upload at https://github.com/pypa/warehouse/blob/master/warehouse/forklift/legacy.py#L798-L803 and the relevant line is `(Release.version == form.version.data)).one()` (assuming the version strings match, not equivalences like `4.1` == `4.1.0`).
Yea, in addition to the "display" version (that keeps track of what the author actually put) we should probably add a normalized version column which is part of the uniqueness of the table, which does things like strip padded zeros and the like.
I feel like this is something the `packaging` module should be able to do, so I made an issue: https://github.com/pypa/packaging/issues/121
@brainwane Yes, it was a 500. | 2018-02-15T23:39:10Z | [] | [] |
pypi/warehouse | 2,959 | pypi__warehouse-2959 | [
"999",
"2831"
] | 9ef365393b11d0f95ff85615a8545e224984c328 | diff --git a/warehouse/accounts/forms.py b/warehouse/accounts/forms.py
--- a/warehouse/accounts/forms.py
+++ b/warehouse/accounts/forms.py
@@ -78,10 +78,25 @@ class PasswordMixin:
],
)
+ def validate_password(self, field):
+ userid = self.user_service.find_userid(self.username.data)
+ if userid is not None:
+ try:
+ if not self.user_service.check_password(userid, field.data):
+ raise wtforms.validators.ValidationError(
+ "The password you have provided is invalid. Please "
+ "try again."
+ )
+ except TooManyFailedLogins:
+ raise wtforms.validators.ValidationError(
+ "There have been too many unsuccessful login attempts, "
+ "please try again later."
+ ) from None
+
class NewPasswordMixin:
- password = wtforms.PasswordField(
+ new_password = wtforms.PasswordField(
validators=[
wtforms.validators.DataRequired(),
forms.PasswordStrengthValidator(
@@ -94,11 +109,23 @@ class NewPasswordMixin:
validators=[
wtforms.validators.DataRequired(),
wtforms.validators.EqualTo(
- "password", "Your passwords do not match. Please try again."
+ "new_password",
+ "Your passwords do not match. Please try again."
),
],
)
+ # These fields are here to provide the various user-defined fields to the
+ # PasswordStrengthValidator of the new_password field, to ensure that the
+ # newly set password doesn't contain any of them
+ full_name = wtforms.StringField() # May be empty
+ username = wtforms.StringField(validators=[
+ wtforms.validators.DataRequired(),
+ ])
+ email = wtforms.StringField(validators=[
+ wtforms.validators.DataRequired(),
+ ])
+
class NewEmailMixin:
@@ -129,7 +156,7 @@ def validate_email(self, field):
class RegistrationForm(
- NewPasswordMixin, NewUsernameMixin, NewEmailMixin, forms.Form):
+ NewUsernameMixin, NewEmailMixin, NewPasswordMixin, forms.Form):
full_name = wtforms.StringField()
g_recaptcha_response = wtforms.StringField()
@@ -157,21 +184,6 @@ def __init__(self, *args, user_service, **kwargs):
super().__init__(*args, **kwargs)
self.user_service = user_service
- def validate_password(self, field):
- userid = self.user_service.find_userid(self.username.data)
- if userid is not None:
- try:
- if not self.user_service.check_password(userid, field.data):
- raise wtforms.validators.ValidationError(
- "The username and password combination you have "
- "provided is invalid. Please try again."
- )
- except TooManyFailedLogins:
- raise wtforms.validators.ValidationError(
- "There have been too many unsuccessful login attempts, "
- "please try again later."
- ) from None
-
class RequestPasswordResetForm(UsernameMixin, forms.Form):
@@ -182,9 +194,4 @@ def __init__(self, *args, user_service, **kwargs):
class ResetPasswordForm(NewPasswordMixin, forms.Form):
- # These fields are here to provide the various user-defined fields to the
- # PasswordStrengthValidator of the NewPasswordMixin, to ensure that the
- # newly set password doesn't contain any of them
- full_name = wtforms.StringField()
- username = wtforms.StringField()
- email = wtforms.StringField()
+ pass
diff --git a/warehouse/accounts/views.py b/warehouse/accounts/views.py
--- a/warehouse/accounts/views.py
+++ b/warehouse/accounts/views.py
@@ -242,7 +242,7 @@ def register(request, _form_class=RegistrationForm):
if request.method == "POST" and form.validate():
user = user_service.create_user(
- form.username.data, form.full_name.data, form.password.data
+ form.username.data, form.full_name.data, form.new_password.data,
)
email = user_service.add_email(user.id, form.email.data, primary=True)
@@ -328,7 +328,7 @@ def _error(message):
)
form = _form_class(
- request.params,
+ **request.params,
username=user.username,
full_name=user.name,
email=user.email,
@@ -337,7 +337,7 @@ def _error(message):
if request.method == "POST" and form.validate():
# Update password.
- user_service.update_user(user.id, password=form.password.data)
+ user_service.update_user(user.id, password=form.new_password.data)
# Flash a success message
request.session.flash(
diff --git a/warehouse/email.py b/warehouse/email.py
--- a/warehouse/email.py
+++ b/warehouse/email.py
@@ -51,15 +51,11 @@ def send_password_reset_email(request, user):
}
subject = render(
- 'email/password-reset.subject.txt',
- fields,
- request=request,
+ 'email/password-reset.subject.txt', fields, request=request
)
body = render(
- 'email/password-reset.body.txt',
- fields,
- request=request,
+ 'email/password-reset.body.txt', fields, request=request
)
request.task(send_email).delay(body, [user.email], subject)
@@ -84,17 +80,31 @@ def send_email_verification_email(request, email):
}
subject = render(
- 'email/verify-email.subject.txt',
- fields,
- request=request,
+ 'email/verify-email.subject.txt', fields, request=request
)
body = render(
- 'email/verify-email.body.txt',
- fields,
- request=request,
+ 'email/verify-email.body.txt', fields, request=request
)
request.task(send_email).delay(body, [email.email], subject)
return fields
+
+
+def send_password_change_email(request, user):
+ fields = {
+ 'username': user.username,
+ }
+
+ subject = render(
+ 'email/password-change.subject.txt', fields, request=request
+ )
+
+ body = render(
+ 'email/password-change.body.txt', fields, request=request
+ )
+
+ request.task(send_email).delay(body, [user.email], subject)
+
+ return fields
diff --git a/warehouse/manage/forms.py b/warehouse/manage/forms.py
--- a/warehouse/manage/forms.py
+++ b/warehouse/manage/forms.py
@@ -13,7 +13,9 @@
import wtforms
from warehouse import forms
-from warehouse.accounts.forms import NewEmailMixin
+from warehouse.accounts.forms import (
+ NewEmailMixin, NewPasswordMixin, PasswordMixin,
+)
class RoleNameMixin:
@@ -72,3 +74,12 @@ class AddEmailForm(NewEmailMixin, forms.Form):
def __init__(self, *args, user_service, **kwargs):
super().__init__(*args, **kwargs)
self.user_service = user_service
+
+
+class ChangePasswordForm(PasswordMixin, NewPasswordMixin, forms.Form):
+
+ __params__ = ['password', 'new_password', 'password_confirm']
+
+ def __init__(self, *args, user_service, **kwargs):
+ super().__init__(*args, **kwargs)
+ self.user_service = user_service
diff --git a/warehouse/manage/views.py b/warehouse/manage/views.py
--- a/warehouse/manage/views.py
+++ b/warehouse/manage/views.py
@@ -20,9 +20,12 @@
from warehouse.accounts.interfaces import IUserService
from warehouse.accounts.models import User, Email
-from warehouse.email import send_email_verification_email
+from warehouse.email import (
+ send_email_verification_email, send_password_change_email,
+)
from warehouse.manage.forms import (
- AddEmailForm, CreateRoleForm, ChangeRoleForm, SaveProfileForm
+ AddEmailForm, ChangePasswordForm, CreateRoleForm, ChangeRoleForm,
+ SaveProfileForm,
)
from warehouse.packaging.models import JournalEntry, Role, File
from warehouse.utils.project import confirm_project, remove_project
@@ -46,6 +49,9 @@ def default_response(self):
return {
'save_profile_form': SaveProfileForm(name=self.request.user.name),
'add_email_form': AddEmailForm(user_service=self.user_service),
+ 'change_password_form': ChangePasswordForm(
+ user_service=self.user_service
+ ),
}
@view_config(request_method="GET")
@@ -184,6 +190,34 @@ def reverify_email(self):
return self.default_response
+ @view_config(
+ request_method='POST',
+ request_param=ChangePasswordForm.__params__,
+ )
+ def change_password(self):
+ form = ChangePasswordForm(
+ **self.request.POST,
+ username=self.request.user.username,
+ full_name=self.request.user.name,
+ email=self.request.user.email,
+ user_service=self.user_service,
+ )
+
+ if form.validate():
+ self.user_service.update_user(
+ self.request.user.id,
+ password=form.new_password.data,
+ )
+ send_password_change_email(self.request, self.request.user)
+ self.request.session.flash(
+ 'Password updated.', queue='success'
+ )
+
+ return {
+ **self.default_response,
+ 'change_password_form': form,
+ }
+
@view_config(
route_name="manage.projects",
| diff --git a/tests/unit/accounts/test_forms.py b/tests/unit/accounts/test_forms.py
--- a/tests/unit/accounts/test_forms.py
+++ b/tests/unit/accounts/test_forms.py
@@ -158,7 +158,7 @@ def test_passwords_mismatch_error(self):
)
form = forms.RegistrationForm(
data={
- "password": "password",
+ "new_password": "password",
"password_confirm": "mismatch",
},
user_service=user_service,
@@ -179,7 +179,7 @@ def test_passwords_match_success(self):
)
form = forms.RegistrationForm(
data={
- "password": "MyStr0ng!shPassword",
+ "new_password": "MyStr0ng!shPassword",
"password_confirm": "MyStr0ng!shPassword",
},
user_service=user_service,
@@ -187,7 +187,7 @@ def test_passwords_match_success(self):
)
form.validate()
- assert len(form.password.errors) == 0
+ assert len(form.new_password.errors) == 0
assert len(form.password_confirm.errors) == 0
def test_email_required_error(self):
@@ -342,7 +342,7 @@ def test_password_strength(self):
)
for pwd, valid in cases:
form = forms.RegistrationForm(
- data={"password": pwd, "password_confirm": pwd},
+ data={"new_password": pwd, "password_confirm": pwd},
user_service=pretend.stub(),
recaptcha_service=pretend.stub(
enabled=False,
@@ -350,7 +350,7 @@ def test_password_strength(self):
),
)
form.validate()
- assert (len(form.password.errors) == 0) == valid
+ assert (len(form.new_password.errors) == 0) == valid
class TestRequestPasswordResetForm:
@@ -400,8 +400,11 @@ def test_password_confirm_required_error(self):
def test_passwords_mismatch_error(self):
form = forms.ResetPasswordForm(
data={
- "password": "password",
+ "new_password": "password",
"password_confirm": "mismatch",
+ "username": "username",
+ "full_name": "full_name",
+ "email": "email",
},
)
@@ -418,15 +421,25 @@ def test_passwords_mismatch_error(self):
])
def test_password_strength(self, password, expected):
form = forms.ResetPasswordForm(
- data={"password": password, "password_confirm": password},
+ data={
+ "new_password": password,
+ "password_confirm": password,
+ "username": "username",
+ "full_name": "full_name",
+ "email": "email",
+ },
)
+
assert form.validate() == expected
def test_passwords_match_success(self):
form = forms.ResetPasswordForm(
data={
- "password": "MyStr0ng!shPassword",
+ "new_password": "MyStr0ng!shPassword",
"password_confirm": "MyStr0ng!shPassword",
+ "username": "username",
+ "full_name": "full_name",
+ "email": "email",
},
)
diff --git a/tests/unit/accounts/test_views.py b/tests/unit/accounts/test_views.py
--- a/tests/unit/accounts/test_views.py
+++ b/tests/unit/accounts/test_views.py
@@ -330,7 +330,7 @@ def test_register_redirect(self, db_request, monkeypatch):
db_request.route_path = pretend.call_recorder(lambda name: "/")
db_request.POST.update({
"username": "username_value",
- "password": "MyStr0ng!shP455w0rd",
+ "new_password": "MyStr0ng!shP455w0rd",
"password_confirm": "MyStr0ng!shP455w0rd",
"email": "[email protected]",
"full_name": "full_name",
@@ -481,7 +481,7 @@ def test_get(self, db_request, user_service, token_service):
assert result["form"] is form_inst
assert form_class.calls == [
pretend.call(
- db_request.GET,
+ token=db_request.params['token'],
username=user.username,
full_name=user.name,
email=user.email,
@@ -501,7 +501,7 @@ def test_reset_password(self, db_request, user_service, token_service):
db_request.method = "POST"
db_request.POST.update({"token": "RANDOM_KEY"})
form_obj = pretend.stub(
- password=pretend.stub(data="password_value"),
+ new_password=pretend.stub(data="password_value"),
validate=pretend.call_recorder(lambda *args: True)
)
@@ -537,7 +537,7 @@ def test_reset_password(self, db_request, user_service, token_service):
assert form_obj.validate.calls == [pretend.call()]
assert form_class.calls == [
pretend.call(
- db_request.POST,
+ token=db_request.params['token'],
username=user.username,
full_name=user.name,
email=user.email,
@@ -549,7 +549,7 @@ def test_reset_password(self, db_request, user_service, token_service):
pretend.call('RANDOM_KEY'),
]
assert user_service.update_user.calls == [
- pretend.call(user.id, password=form_obj.password.data),
+ pretend.call(user.id, password=form_obj.new_password.data),
pretend.call(user.id, last_login=now),
]
assert db_request.session.flash.calls == [
diff --git a/tests/unit/manage/test_forms.py b/tests/unit/manage/test_forms.py
--- a/tests/unit/manage/test_forms.py
+++ b/tests/unit/manage/test_forms.py
@@ -78,3 +78,12 @@ def test_creation(self):
form = forms.AddEmailForm(user_service=user_service)
assert form.user_service is user_service
+
+
+class TestChangePasswordForm:
+
+ def test_creation(self):
+ user_service = pretend.stub()
+ form = forms.ChangePasswordForm(user_service=user_service)
+
+ assert form.user_service is user_service
diff --git a/tests/unit/manage/test_views.py b/tests/unit/manage/test_views.py
--- a/tests/unit/manage/test_views.py
+++ b/tests/unit/manage/test_views.py
@@ -42,14 +42,21 @@ def test_default_response(self, monkeypatch):
save_profile_obj = pretend.stub()
save_profile_cls = pretend.call_recorder(lambda **kw: save_profile_obj)
monkeypatch.setattr(views, 'SaveProfileForm', save_profile_cls)
+
add_email_obj = pretend.stub()
- add_email_cls = pretend.call_recorder(lambda *a, **kw: add_email_obj)
+ add_email_cls = pretend.call_recorder(lambda **kw: add_email_obj)
monkeypatch.setattr(views, 'AddEmailForm', add_email_cls)
+
+ change_pass_obj = pretend.stub()
+ change_pass_cls = pretend.call_recorder(lambda **kw: change_pass_obj)
+ monkeypatch.setattr(views, 'ChangePasswordForm', change_pass_cls)
+
view = views.ManageProfileViews(request)
assert view.default_response == {
'save_profile_form': save_profile_obj,
'add_email_form': add_email_obj,
+ 'change_password_form': change_pass_obj,
}
assert view.request == request
assert view.user_service == user_service
@@ -59,6 +66,9 @@ def test_default_response(self, monkeypatch):
assert add_email_cls.calls == [
pretend.call(user_service=user_service),
]
+ assert change_pass_cls.calls == [
+ pretend.call(user_service=user_service),
+ ]
def test_manage_profile(self, monkeypatch):
user_service = pretend.stub()
@@ -379,7 +389,7 @@ def test_reverify_email(self, monkeypatch):
flash=pretend.call_recorder(lambda *a, **kw: None)
),
find_service=lambda *a, **kw: pretend.stub(),
- user=pretend.stub(id=pretend.stub),
+ user=pretend.stub(id=pretend.stub()),
)
send_email = pretend.call_recorder(lambda *a: None)
monkeypatch.setattr(views, 'send_email_verification_email', send_email)
@@ -412,7 +422,7 @@ def raise_no_result():
flash=pretend.call_recorder(lambda *a, **kw: None)
),
find_service=lambda *a, **kw: pretend.stub(),
- user=pretend.stub(id=pretend.stub),
+ user=pretend.stub(id=pretend.stub()),
)
send_email = pretend.call_recorder(lambda *a: None)
monkeypatch.setattr(views, 'send_email_verification_email', send_email)
@@ -441,7 +451,7 @@ def test_reverify_email_already_verified(self, monkeypatch):
flash=pretend.call_recorder(lambda *a, **kw: None)
),
find_service=lambda *a, **kw: pretend.stub(),
- user=pretend.stub(id=pretend.stub),
+ user=pretend.stub(id=pretend.stub()),
)
send_email = pretend.call_recorder(lambda *a: None)
monkeypatch.setattr(views, 'send_email_verification_email', send_email)
@@ -456,6 +466,100 @@ def test_reverify_email_already_verified(self, monkeypatch):
]
assert send_email.calls == []
+ def test_change_password(self, monkeypatch):
+ old_password = '0ld_p455w0rd'
+ new_password = 'n3w_p455w0rd'
+ user_service = pretend.stub(
+ update_user=pretend.call_recorder(lambda *a, **kw: None)
+ )
+ request = pretend.stub(
+ POST={
+ 'password': old_password,
+ 'new_password': new_password,
+ 'password_confirm': new_password,
+ },
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None)
+ ),
+ find_service=lambda *a, **kw: user_service,
+ user=pretend.stub(
+ id=pretend.stub(),
+ username=pretend.stub(),
+ email=pretend.stub(),
+ name=pretend.stub(),
+ ),
+ )
+ change_pwd_obj = pretend.stub(
+ validate=lambda: True,
+ new_password=pretend.stub(data=new_password),
+ )
+ change_pwd_cls = pretend.call_recorder(lambda *a, **kw: change_pwd_obj)
+ monkeypatch.setattr(views, 'ChangePasswordForm', change_pwd_cls)
+
+ send_email = pretend.call_recorder(lambda *a: None)
+ monkeypatch.setattr(views, 'send_password_change_email', send_email)
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'default_response', {'_': pretend.stub()}
+ )
+ view = views.ManageProfileViews(request)
+
+ assert view.change_password() == {
+ **view.default_response,
+ 'change_password_form': change_pwd_obj,
+ }
+ assert request.session.flash.calls == [
+ pretend.call('Password updated.', queue='success'),
+ ]
+ assert send_email.calls == [pretend.call(request, request.user)]
+ assert user_service.update_user.calls == [
+ pretend.call(request.user.id, password=new_password),
+ ]
+
+ def test_change_password_validation_fails(self, monkeypatch):
+ old_password = '0ld_p455w0rd'
+ new_password = 'n3w_p455w0rd'
+ user_service = pretend.stub(
+ update_user=pretend.call_recorder(lambda *a, **kw: None)
+ )
+ request = pretend.stub(
+ POST={
+ 'password': old_password,
+ 'new_password': new_password,
+ 'password_confirm': new_password,
+ },
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None)
+ ),
+ find_service=lambda *a, **kw: user_service,
+ user=pretend.stub(
+ id=pretend.stub(),
+ username=pretend.stub(),
+ email=pretend.stub(),
+ name=pretend.stub(),
+ ),
+ )
+ change_pwd_obj = pretend.stub(
+ validate=lambda: False,
+ new_password=pretend.stub(data=new_password),
+ )
+ change_pwd_cls = pretend.call_recorder(lambda *a, **kw: change_pwd_obj)
+ monkeypatch.setattr(views, 'ChangePasswordForm', change_pwd_cls)
+
+ send_email = pretend.call_recorder(lambda *a: None)
+ monkeypatch.setattr(views, 'send_password_change_email', send_email)
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'default_response', {'_': pretend.stub()}
+ )
+ view = views.ManageProfileViews(request)
+
+ assert view.change_password() == {
+ **view.default_response,
+ 'change_password_form': change_pwd_obj,
+ }
+ assert request.session.flash.calls == []
+ assert send_email.calls == []
+ assert user_service.update_user.calls == []
+
class TestManageProjects:
diff --git a/tests/unit/test_email.py b/tests/unit/test_email.py
--- a/tests/unit/test_email.py
+++ b/tests/unit/test_email.py
@@ -217,3 +217,47 @@ def test_email_verification_email(
assert send_email.delay.calls == [
pretend.call('Email Body', [stub_email.email], 'Email Subject'),
]
+
+
+class TestPasswordChangeEmail:
+
+ def test_password_change_email(
+ self, pyramid_request, pyramid_config, monkeypatch):
+
+ stub_user = pretend.stub(
+ email='email',
+ username='username',
+ )
+ subject_renderer = pyramid_config.testing_add_renderer(
+ 'email/password-change.subject.txt'
+ )
+ subject_renderer.string_response = 'Email Subject'
+ body_renderer = pyramid_config.testing_add_renderer(
+ 'email/password-change.body.txt'
+ )
+ body_renderer.string_response = 'Email Body'
+
+ send_email = pretend.stub(
+ delay=pretend.call_recorder(lambda *args, **kwargs: None)
+ )
+ pyramid_request.task = pretend.call_recorder(
+ lambda *args, **kwargs: send_email
+ )
+ monkeypatch.setattr(email, 'send_email', send_email)
+
+ result = email.send_password_change_email(
+ pyramid_request,
+ user=stub_user,
+ )
+
+ assert result == {
+ 'username': stub_user.username,
+ }
+ subject_renderer.assert_()
+ body_renderer.assert_(username=stub_user.username)
+ assert pyramid_request.task.calls == [
+ pretend.call(send_email),
+ ]
+ assert send_email.delay.calls == [
+ pretend.call('Email Body', [stub_user.email], 'Email Subject'),
+ ]
| Send confirmation email when password changed
Currently on PyPI, if a user changes their password, there is no email notification, meaning account compromise can go unnoticed.
Changing of password doesn't seem to be implemented on https://warehouse.python.org/ yet, but filing this now to ensure it's not forgotten :-)
Manage Account: Change Password
From #423: From the 'manage account' page, a logged-in user should be able to change their password by providing:
* their current password
* a new password
* confirmation for their new password
A notification email should be sent to the primary email address when the password is successfully changed.
| (And for that matter, I really hope the new implementation will require that the user confirm their existing password, rather than just allow changing it via the session cookie.)
| 2018-02-16T17:33:29Z | [] | [] |
pypi/warehouse | 2,960 | pypi__warehouse-2960 | [
"2065"
] | 5b436174b207361541d29b3a50f263d9e53c0e13 | diff --git a/warehouse/accounts/interfaces.py b/warehouse/accounts/interfaces.py
--- a/warehouse/accounts/interfaces.py
+++ b/warehouse/accounts/interfaces.py
@@ -35,7 +35,7 @@ class TokenMissing(Exception):
class IUserService(Interface):
- def get_user(userid):
+ def get_user(user_id):
"""
Return the user object that represents the given userid, or None if
there is no user for that ID.
@@ -53,14 +53,15 @@ def find_userid(username):
is no user with the given username.
"""
- def check_password(userid, password):
+ def check_password(user_id, password):
"""
Returns a boolean representing whether the given password is valid for
the given userid.
"""
- def create_user(username, name, password, email,
- is_active=False, is_staff=False, is_superuser=False):
+ def create_user(
+ username, name, password,
+ is_active=False, is_staff=False, is_superuser=False):
"""
Accepts a user object, and attempts to create a user with those
attributes.
@@ -68,6 +69,11 @@ def create_user(username, name, password, email,
A UserAlreadyExists Exception is raised if the user already exists.
"""
+ def add_email(user_id, email_address, primary=False, verified=False):
+ """
+ Adds an email for the provided user_id
+ """
+
def update_user(user_id, **changes):
"""
Updates the user object
diff --git a/warehouse/accounts/services.py b/warehouse/accounts/services.py
--- a/warehouse/accounts/services.py
+++ b/warehouse/accounts/services.py
@@ -148,8 +148,9 @@ def check_password(self, userid, password):
return False
- def create_user(self, username, name, password, email,
- is_active=False, is_staff=False, is_superuser=False):
+ def create_user(
+ self, username, name, password,
+ is_active=False, is_staff=False, is_superuser=False):
user = User(username=username,
name=name,
@@ -158,13 +159,20 @@ def create_user(self, username, name, password, email,
is_staff=is_staff,
is_superuser=is_superuser)
self.db.add(user)
- email_object = Email(email=email, user=user,
- primary=True, verified=False)
- self.db.add(email_object)
- # flush the db now so user.id is available
- self.db.flush()
+ self.db.flush() # flush the db now so user.id is available
+
return user
+ def add_email(self, user_id, email_address, primary=False, verified=False):
+ user = self.get_user(user_id)
+ email= Email(
+ email=email_address, user=user, primary=primary, verified=verified,
+ )
+ self.db.add(email)
+ self.db.flush() # flush the db now so email.id is available
+
+ return email
+
def update_user(self, user_id, **changes):
user = self.get_user(user_id)
for attr, value in changes.items():
diff --git a/warehouse/accounts/views.py b/warehouse/accounts/views.py
--- a/warehouse/accounts/views.py
+++ b/warehouse/accounts/views.py
@@ -32,7 +32,9 @@
)
from warehouse.accounts.models import Email
from warehouse.cache.origin import origin_cache
-from warehouse.email import send_password_reset_email
+from warehouse.email import (
+ send_password_reset_email, send_email_verification_email,
+)
from warehouse.packaging.models import Project, Release
from warehouse.utils.http import is_safe_url
@@ -231,13 +233,16 @@ def register(request, _form_class=RegistrationForm):
if request.method == "POST" and form.validate():
user = user_service.create_user(
- form.username.data, form.full_name.data, form.password.data,
- form.email.data
+ form.username.data, form.full_name.data, form.password.data
)
+ email = user_service.add_email(user.id, form.email.data, primary=True)
+
+ send_email_verification_email(request, email)
return HTTPSeeOther(
request.route_path("index"),
- headers=dict(_login_user(request, user.id)))
+ headers=dict(_login_user(request, user.id))
+ )
return {"form": form}
diff --git a/warehouse/manage/views.py b/warehouse/manage/views.py
--- a/warehouse/manage/views.py
+++ b/warehouse/manage/views.py
@@ -78,14 +78,9 @@ def add_email(self):
form = AddEmailForm(self.request.POST, user_service=self.user_service)
if form.validate():
- email = Email(
- email=form.email.data,
- user_id=self.request.user.id,
- primary=False,
- verified=False,
+ email = self.user_service.add_email(
+ self.request.user.id, form.email.data,
)
- self.request.user.emails.append(email)
- self.request.db.flush() # To get the new ID
send_email_verification_email(self.request, email)
| diff --git a/tests/unit/accounts/test_services.py b/tests/unit/accounts/test_services.py
--- a/tests/unit/accounts/test_services.py
+++ b/tests/unit/accounts/test_services.py
@@ -140,18 +140,26 @@ def test_check_password_updates(self, user_service):
def test_create_user(self, user_service):
user = UserFactory.build()
- email = "[email protected]"
new_user = user_service.create_user(
username=user.username,
name=user.name,
password=user.password,
- email=email
)
user_service.db.flush()
user_from_db = user_service.get_user(new_user.id)
+
assert user_from_db.username == user.username
assert user_from_db.name == user.name
- assert user_from_db.email == email
+
+ def test_add_email(self, user_service):
+ user = UserFactory.create()
+ email = "[email protected]"
+ new_email = user_service.add_email(user.id, email)
+
+ assert new_email.email == email
+ assert new_email.user == user
+ assert not new_email.primary
+ assert not new_email.verified
def test_update_user(self, user_service):
user = UserFactory.create()
@@ -176,7 +184,8 @@ def test_find_by_email_not_found(self, user_service):
def test_create_login_success(self, user_service):
user = user_service.create_user(
- "test_user", "test_name", "test_password", "test_email")
+ "test_user", "test_name", "test_password",
+ )
assert user.id is not None
# now make sure that we can log in as that user
@@ -184,7 +193,8 @@ def test_create_login_success(self, user_service):
def test_create_login_error(self, user_service):
user = user_service.create_user(
- "test_user", "test_name", "test_password", "test_email")
+ "test_user", "test_name", "test_password",
+ )
assert user.id is not None
assert not user_service.check_password(user.id, "bad_password")
diff --git a/tests/unit/accounts/test_views.py b/tests/unit/accounts/test_views.py
--- a/tests/unit/accounts/test_views.py
+++ b/tests/unit/accounts/test_views.py
@@ -306,8 +306,13 @@ def test_redirect_authenticated_user(self):
assert isinstance(result, HTTPSeeOther)
assert result.headers["Location"] == "/"
- def test_register_redirect(self, pyramid_request):
+ def test_register_redirect(self, pyramid_request, monkeypatch):
pyramid_request.method = "POST"
+
+ user = pretend.stub(id=pretend.stub())
+ email = pretend.stub()
+ create_user = pretend.call_recorder(lambda *args, **kwargs: user)
+ add_email = pretend.call_recorder(lambda *args, **kwargs: email)
pyramid_request.find_service = pretend.call_recorder(
lambda *args, **kwargs: pretend.stub(
csp_policy={},
@@ -316,10 +321,9 @@ def test_register_redirect(self, pyramid_request):
verify_response=pretend.call_recorder(lambda _: None),
find_userid=pretend.call_recorder(lambda _: None),
find_userid_by_email=pretend.call_recorder(lambda _: None),
- create_user=pretend.call_recorder(
- lambda *args, **kwargs: pretend.stub(id=1),
- ),
update_user=lambda *args, **kwargs: None,
+ create_user=create_user,
+ add_email=add_email,
)
)
pyramid_request.route_path = pretend.call_recorder(lambda name: "/")
@@ -330,10 +334,20 @@ def test_register_redirect(self, pyramid_request):
"email": "[email protected]",
"full_name": "full_name",
})
+ send_email = pretend.call_recorder(lambda *a: None)
+ monkeypatch.setattr(views, 'send_email_verification_email', send_email)
result = views.register(pyramid_request)
+
assert isinstance(result, HTTPSeeOther)
assert result.headers["Location"] == "/"
+ assert create_user.calls == [
+ pretend.call('username_value', 'full_name', 'MyStr0ng!shP455w0rd'),
+ ]
+ assert add_email.calls == [
+ pretend.call(user.id, '[email protected]', primary=True),
+ ]
+ assert send_email.calls == [pretend.call(pyramid_request, email)]
class TestRequestPasswordReset:
diff --git a/tests/unit/manage/test_views.py b/tests/unit/manage/test_views.py
--- a/tests/unit/manage/test_views.py
+++ b/tests/unit/manage/test_views.py
@@ -138,7 +138,10 @@ def test_save_profile_validation_fails(self, monkeypatch):
def test_add_email(self, monkeypatch, pyramid_config):
email_address = "[email protected]"
- user_service = pretend.stub()
+ email = pretend.stub(id=pretend.stub(), email=email_address)
+ user_service = pretend.stub(
+ add_email=pretend.call_recorder(lambda *a, **kw: email)
+ )
request = pretend.stub(
POST={'email': email_address},
db=pretend.stub(flush=lambda: None),
@@ -158,10 +161,6 @@ def test_add_email(self, monkeypatch, pyramid_config):
)
)
- email_obj = pretend.stub(id=pretend.stub(), email=email_address)
- email_cls = pretend.call_recorder(lambda **kw: email_obj)
- monkeypatch.setattr(views, 'Email', email_cls)
-
send_email = pretend.call_recorder(lambda *a: None)
monkeypatch.setattr(views, 'send_email_verification_email', send_email)
@@ -171,14 +170,8 @@ def test_add_email(self, monkeypatch, pyramid_config):
view = views.ManageProfileViews(request)
assert view.add_email() == view.default_response
- assert request.user.emails == [email_obj]
- assert email_cls.calls == [
- pretend.call(
- email=email_address,
- user_id=request.user.id,
- primary=False,
- verified=False,
- ),
+ assert user_service.add_email.calls == [
+ pretend.call(request.user.id, email_address)
]
assert request.session.flash.calls == [
pretend.call(
@@ -188,7 +181,7 @@ def test_add_email(self, monkeypatch, pyramid_config):
),
]
assert send_email.calls == [
- pretend.call(request, email_obj),
+ pretend.call(request, email),
]
def test_add_email_validation_fails(self, monkeypatch):
| Send confirmation link in email on new account registration
I just wrote my first package that I wanted to publish on PyPI and didn't have an account. I have registered yesterday through the new registration form: https://pypi.org/account/register/.
The registration process had no issues, the account was directly active and the log in worked. Today I prepared the package for upload but i received a 401 error:
`Upload failed (401): You must be identified to edit package information`
After some research, I saw also other people complaining, and this might happen if the activation link in the email is not clicked. So one problem might be that the activation email is not being sent and the account is directly active.
Since I don't found any way resending an activation link, and the login with the account worked normally I have deleted the account and made another registration using the old PyPI website: https://pypi.python.org/pypi?%3Aaction=register_form
This time I received an activation link, which led me to a page where I also had to accept the submission terms. So probably this part is missing and this is why the authorization failed with the other account.
| Hello, I think the same fix needs to happen for test pypi. The [guide](https://packaging.python.org/guides/using-testpypi/) asks you to register with a link to [https://test.pypi.org/account/register/](https://test.pypi.org/account/register/). I was getting some [`401` responses as well](https://stackoverflow.com/q/29350703/3814202), and then found this issue. Using the linked site, I never received a confirmation e-mail.
**Edit**: see [comment below](https://github.com/pypa/warehouse/issues/2065#issuecomment-329855965) and try that **first**. I suspect my "solution" is invalid, since I was able to "register" but still cannot upload.
Solution: I went to [https://testpypi.python.org/pypi](https://testpypi.python.org/pypi) (where the difference is a `.python` after `testpypi`), deleted the account I just made, and re-registered using this site (so following the link to [https://testpypi.python.org/pypi?%3Aaction=register_form](https://testpypi.python.org/pypi?%3Aaction=register_form)) and saw both a terms agreement as well as received a confirmation e-mail letting me finish the registration.
I believe the [https://github.com/pypa/python-packaging-user-guide](https://github.com/pypa/python-packaging-user-guide) repo is the source code, would a PR fixing the `testpypi` URL be helpful or is the problem more complicated?
I still can't seem to find the right URL to use though. It's either [these issues](https://github.com/pypa/twine/issues/200) or using what I think the right URL should be:
```
Uploading distributions to https://test.pypi.io/legacy
Uploading exhale-0.1.2.tar.gz
RedirectDetected: "https://test.pypi.io/legacy" attempted to redirect to "https://test.pypi.org/legacy" during upload. Aborting...
```
redirect to itself fails?
I can confirm the reported behavior. I just recently wrote up my learnings from publishing a package to PyPI for the first time ([link to my blog post](https://jonemo.github.io/neubertify/2017/09/13/publishing-your-first-pypi-package/)) and found that
* The registration form on test.pypi.org seems to work
* ... but the confirmation email never arrives and there is no indication to the user that one should arrive
* ... therefore users never get to see the terms and cannot activate their account
* ... and then get a confusing error message when attempting to upload a package
The workaround is to use the registration form on pypi.python.org/pypi, but then complete all other steps on pypi.org.
@svenevs: This is my `.pypirc` with URLs for Test PyPI and real PyPI that worked for me:
```
#~/.pypirc
[distutils]
index-servers =
pypi
pypitest
[pypi]
repository: https://pypi.org/legacy/
username: YOUR_USERNAME_GOES_HERE
[pypitest]
repository: https://test.pypi.org/legacy/
username: YOUR_USERNAME_GOES_HERE
```
> The workaround is to use the registration form on pypi.python.org/pypi, but then complete all other steps on pypi.org.
Interesting. It doesn't work for me, I was already registered on "real" pypi before getting the e-mail from the way I went about it (delete, alternative url). As such, the URL you have gives me `403` errors :S Hopefully your fix will apply to most situations. I think I pigeonholed myself somehow. | 2018-02-16T18:41:23Z | [] | [] |
pypi/warehouse | 2,978 | pypi__warehouse-2978 | [
"2832"
] | f8d132cb45eec7e34e04ac73b677700523e1a5b2 | diff --git a/warehouse/email.py b/warehouse/email.py
--- a/warehouse/email.py
+++ b/warehouse/email.py
@@ -108,3 +108,21 @@ def send_password_change_email(request, user):
request.task(send_email).delay(body, [user.email], subject)
return fields
+
+
+def send_account_deletion_email(request, user):
+ fields = {
+ 'username': user.username,
+ }
+
+ subject = render(
+ 'email/account-deleted.subject.txt', fields, request=request
+ )
+
+ body = render(
+ 'email/account-deleted.body.txt', fields, request=request
+ )
+
+ request.task(send_email).delay(body, [user.email], subject)
+
+ return fields
diff --git a/warehouse/manage/views.py b/warehouse/manage/views.py
--- a/warehouse/manage/views.py
+++ b/warehouse/manage/views.py
@@ -1,5 +1,4 @@
# Licensed under the Apache License, Version 2.0 (the "License");
-
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
@@ -16,18 +15,21 @@
from pyramid.httpexceptions import HTTPSeeOther
from pyramid.security import Authenticated
from pyramid.view import view_config, view_defaults
+from sqlalchemy import func
from sqlalchemy.orm.exc import NoResultFound
from warehouse.accounts.interfaces import IUserService
from warehouse.accounts.models import User, Email
+from warehouse.accounts.views import logout
from warehouse.email import (
- send_email_verification_email, send_password_change_email,
+ send_account_deletion_email, send_email_verification_email,
+ send_password_change_email,
)
from warehouse.manage.forms import (
AddEmailForm, ChangePasswordForm, CreateRoleForm, ChangeRoleForm,
SaveProfileForm,
)
-from warehouse.packaging.models import JournalEntry, Role, File
+from warehouse.packaging.models import File, JournalEntry, Project, Role
from warehouse.utils.project import confirm_project, remove_project
@@ -44,6 +46,32 @@ def __init__(self, request):
self.request = request
self.user_service = request.find_service(IUserService, context=None)
+ @property
+ def active_projects(self):
+ ''' Return all the projects for with the user is a sole owner '''
+ projects_owned = (
+ self.request.db.query(Project)
+ .join(Role.project)
+ .filter(Role.role_name == 'Owner', Role.user == self.request.user)
+ .subquery()
+ )
+
+ with_sole_owner = (
+ self.request.db.query(Role.package_name)
+ .join(projects_owned)
+ .filter(Role.role_name == 'Owner')
+ .group_by(Role.package_name)
+ .having(func.count(Role.package_name) == 1)
+ .subquery()
+ )
+
+ return (
+ self.request.db.query(Project)
+ .join(with_sole_owner)
+ .order_by(Project.name)
+ .all()
+ )
+
@property
def default_response(self):
return {
@@ -52,6 +80,7 @@ def default_response(self):
'change_password_form': ChangePasswordForm(
user_service=self.user_service
),
+ 'active_projects': self.active_projects,
}
@view_config(request_method="GET")
@@ -218,6 +247,58 @@ def change_password(self):
'change_password_form': form,
}
+ @view_config(
+ request_method='POST',
+ request_param=['confirm_username']
+ )
+ def delete_account(self):
+ username = self.request.params.get('confirm_username')
+
+ if not username:
+ self.request.session.flash(
+ "Must confirm the request.", queue='error'
+ )
+ return self.default_response
+
+ if username != self.request.user.username:
+ self.request.session.flash(
+ f"Could not delete account - {username!r} is not the same as "
+ f"{self.request.user.username!r}",
+ queue='error'
+ )
+ return self.default_response
+
+ if self.active_projects:
+ self.request.session.flash(
+ "Cannot delete account with active project ownerships.",
+ queue='error',
+ )
+ return self.default_response
+
+ # Update all journals to point to `deleted-user` instead
+ deleted_user = (
+ self.request.db.query(User)
+ .filter(User.username == 'deleted-user')
+ .one()
+ )
+
+ journals = (
+ self.request.db.query(JournalEntry)
+ .filter(JournalEntry.submitted_by == self.request.user)
+ .all()
+ )
+
+ for journal in journals:
+ journal.submitted_by = deleted_user
+
+ # Send a notification email
+ send_account_deletion_email(self.request, self.request.user)
+
+ # Actually delete the user
+ self.request.db.delete(self.request.user)
+
+ return logout(self.request)
+
@view_config(
route_name="manage.projects",
| diff --git a/tests/unit/manage/test_views.py b/tests/unit/manage/test_views.py
--- a/tests/unit/manage/test_views.py
+++ b/tests/unit/manage/test_views.py
@@ -22,7 +22,7 @@
from warehouse.manage import views
from warehouse.accounts.interfaces import IUserService
-from warehouse.packaging.models import JournalEntry, Project, Role
+from warehouse.packaging.models import JournalEntry, Project, Role, User
from ...common.db.accounts import EmailFactory
from ...common.db.packaging import (
@@ -53,10 +53,15 @@ def test_default_response(self, monkeypatch):
view = views.ManageProfileViews(request)
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'active_projects', pretend.stub()
+ )
+
assert view.default_response == {
'save_profile_form': save_profile_obj,
'add_email_form': add_email_obj,
'change_password_form': change_pass_obj,
+ 'active_projects': view.active_projects,
}
assert view.request == request
assert view.user_service == user_service
@@ -70,6 +75,44 @@ def test_default_response(self, monkeypatch):
pretend.call(user_service=user_service),
]
+ def test_active_projects(self, db_request):
+ user = UserFactory.create()
+ another_user = UserFactory.create()
+
+ db_request.user = user
+ db_request.find_service = lambda *a, **kw: pretend.stub()
+
+ # A project with a sole owner that is the user
+ with_sole_owner = ProjectFactory.create()
+ RoleFactory.create(
+ user=user, project=with_sole_owner, role_name='Owner'
+ )
+ RoleFactory.create(
+ user=another_user, project=with_sole_owner, role_name='Maintainer'
+ )
+
+ # A project with multiple owners, including the user
+ with_multiple_owners = ProjectFactory.create()
+ RoleFactory.create(
+ user=user, project=with_multiple_owners, role_name='Owner'
+ )
+ RoleFactory.create(
+ user=another_user, project=with_multiple_owners, role_name='Owner'
+ )
+
+ # A project with a sole owner that is not the user
+ not_an_owner = ProjectFactory.create()
+ RoleFactory.create(
+ user=user, project=not_an_owner, role_name='Maintatiner'
+ )
+ RoleFactory.create(
+ user=another_user, project=not_an_owner, role_name='Owner'
+ )
+
+ view = views.ManageProfileViews(db_request)
+
+ assert view.active_projects == [with_sole_owner]
+
def test_manage_profile(self, monkeypatch):
user_service = pretend.stub()
name = pretend.stub()
@@ -560,6 +603,105 @@ def test_change_password_validation_fails(self, monkeypatch):
assert send_email.calls == []
assert user_service.update_user.calls == []
+ def test_delete_account(self, monkeypatch, db_request):
+ user = UserFactory.create()
+ deleted_user = UserFactory.create(username='deleted-user')
+ journal = JournalEntryFactory(submitted_by=user)
+
+ db_request.user = user
+ db_request.params = {'confirm_username': user.username}
+ db_request.find_service = lambda *a, **kw: pretend.stub()
+
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'default_response', pretend.stub()
+ )
+ monkeypatch.setattr(views.ManageProfileViews, 'active_projects', [])
+ send_email = pretend.call_recorder(lambda *a: None)
+ monkeypatch.setattr(views, 'send_account_deletion_email', send_email)
+ logout_response = pretend.stub()
+ logout = pretend.call_recorder(lambda *a: logout_response)
+ monkeypatch.setattr(views, 'logout', logout)
+
+ view = views.ManageProfileViews(db_request)
+
+ assert view.delete_account() == logout_response
+ assert journal.submitted_by == deleted_user
+ assert db_request.db.query(User).all() == [deleted_user]
+ assert send_email.calls == [pretend.call(db_request, user)]
+ assert logout.calls == [pretend.call(db_request)]
+
+ def test_delete_account_no_confirm(self, monkeypatch):
+ request = pretend.stub(
+ params={'confirm_username': ''},
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ ),
+ find_service=lambda *a, **kw: pretend.stub(),
+ )
+
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'default_response', pretend.stub()
+ )
+
+ view = views.ManageProfileViews(request)
+
+ assert view.delete_account() == view.default_response
+ assert request.session.flash.calls == [
+ pretend.call('Must confirm the request.', queue='error')
+ ]
+
+ def test_delete_account_wrong_confirm(self, monkeypatch):
+ request = pretend.stub(
+ params={'confirm_username': 'invalid'},
+ user=pretend.stub(username='username'),
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ ),
+ find_service=lambda *a, **kw: pretend.stub(),
+ )
+
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'default_response', pretend.stub()
+ )
+
+ view = views.ManageProfileViews(request)
+
+ assert view.delete_account() == view.default_response
+ assert request.session.flash.calls == [
+ pretend.call(
+ "Could not delete account - 'invalid' is not the same as "
+ "'username'",
+ queue='error',
+ )
+ ]
+
+ def test_delete_account_has_active_projects(self, monkeypatch):
+ request = pretend.stub(
+ params={'confirm_username': 'username'},
+ user=pretend.stub(username='username'),
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ ),
+ find_service=lambda *a, **kw: pretend.stub(),
+ )
+
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'default_response', pretend.stub()
+ )
+ monkeypatch.setattr(
+ views.ManageProfileViews, 'active_projects', [pretend.stub()]
+ )
+
+ view = views.ManageProfileViews(request)
+
+ assert view.delete_account() == view.default_response
+ assert request.session.flash.calls == [
+ pretend.call(
+ "Cannot delete account with active project ownerships.",
+ queue='error',
+ )
+ ]
+
class TestManageProjects:
diff --git a/tests/unit/test_email.py b/tests/unit/test_email.py
--- a/tests/unit/test_email.py
+++ b/tests/unit/test_email.py
@@ -261,3 +261,47 @@ def test_password_change_email(
assert send_email.delay.calls == [
pretend.call('Email Body', [stub_user.email], 'Email Subject'),
]
+
+
+class TestAccountDeletionEmail:
+
+ def test_account_deletion_email(
+ self, pyramid_request, pyramid_config, monkeypatch):
+
+ stub_user = pretend.stub(
+ email='email',
+ username='username',
+ )
+ subject_renderer = pyramid_config.testing_add_renderer(
+ 'email/account-deleted.subject.txt'
+ )
+ subject_renderer.string_response = 'Email Subject'
+ body_renderer = pyramid_config.testing_add_renderer(
+ 'email/account-deleted.body.txt'
+ )
+ body_renderer.string_response = 'Email Body'
+
+ send_email = pretend.stub(
+ delay=pretend.call_recorder(lambda *args, **kwargs: None)
+ )
+ pyramid_request.task = pretend.call_recorder(
+ lambda *args, **kwargs: send_email
+ )
+ monkeypatch.setattr(email, 'send_email', send_email)
+
+ result = email.send_account_deletion_email(
+ pyramid_request,
+ user=stub_user,
+ )
+
+ assert result == {
+ 'username': stub_user.username,
+ }
+ subject_renderer.assert_()
+ body_renderer.assert_(username=stub_user.username)
+ assert pyramid_request.task.calls == [
+ pretend.call(send_email),
+ ]
+ assert send_email.delay.calls == [
+ pretend.call('Email Body', [stub_user.email], 'Email Subject'),
+ ]
| Manage Account: Delete Account
From #423: From the 'manage account' page, a logged-in user should be able to delete their account.
If the user is the sole owner of any projects, this should be disabled and they should be informed that they need to transfer ownership or delete the projects before they can delete their account.
A notification email should be sent to the primary email address when the account is successfully deleted.
| @di
I've started diving into the code and would like to work on this issue.
I'll get back if I get any questions and will put a WIP PR.
Hi @waseem18, I appreciate your enthusiasm! However I've already begun work on this issue. If you're looking for something relevant to work on, I'd recommend searching for issues in upcoming milestones without an assignee. [The project view for this repo might be helpful as well](https://github.com/pypa/warehouse/projects/1).
Thanks for the information @di | 2018-02-19T00:21:54Z | [] | [] |
pypi/warehouse | 2,984 | pypi__warehouse-2984 | [
"2936"
] | 4e0c8c3fd67c7eca4a10c81c350c7d23e2423a9f | diff --git a/warehouse/accounts/views.py b/warehouse/accounts/views.py
--- a/warehouse/accounts/views.py
+++ b/warehouse/accounts/views.py
@@ -103,6 +103,8 @@ def login(request, redirect_field_name=REDIRECT_FIELD_NAME,
# TODO: Logging in should reset request.user
# TODO: Configure the login view as the default view for not having
# permission to view something.
+ if request.authenticated_userid is not None:
+ return HTTPSeeOther(request.route_path('manage.projects'))
user_service = request.find_service(IUserService, context=None)
@@ -213,7 +215,7 @@ def logout(request, redirect_field_name=REDIRECT_FIELD_NAME):
)
def register(request, _form_class=RegistrationForm):
if request.authenticated_userid is not None:
- return HTTPSeeOther("/")
+ return HTTPSeeOther(request.route_path('manage.projects'))
if AdminFlag.is_enabled(request.db, 'disallow-new-user-registration'):
request.session.flash(
| diff --git a/tests/unit/accounts/test_views.py b/tests/unit/accounts/test_views.py
--- a/tests/unit/accounts/test_views.py
+++ b/tests/unit/accounts/test_views.py
@@ -238,6 +238,15 @@ def test_post_validate_no_redirects(self, pyramid_request,
assert isinstance(result, HTTPSeeOther)
assert result.headers["Location"] == observed_next_url
+ def test_redirect_authenticated_user(self):
+ pyramid_request = pretend.stub(authenticated_userid=1)
+ pyramid_request.route_path = pretend.call_recorder(
+ lambda a: '/the-redirect'
+ )
+ result = views.login(pyramid_request)
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/the-redirect"
+
class TestLogout:
@@ -303,9 +312,13 @@ def test_get(self, db_request):
assert result["form"] is form_inst
def test_redirect_authenticated_user(self):
- result = views.register(pretend.stub(authenticated_userid=1))
+ pyramid_request = pretend.stub(authenticated_userid=1)
+ pyramid_request.route_path = pretend.call_recorder(
+ lambda a: '/the-redirect'
+ )
+ result = views.register(pyramid_request)
assert isinstance(result, HTTPSeeOther)
- assert result.headers["Location"] == "/"
+ assert result.headers["Location"] == "/the-redirect"
def test_register_redirect(self, db_request, monkeypatch):
db_request.method = "POST"
| Redirect from login page if user is logged in
Currently the ` accounts.login` view will still render even if there's already user logged in.
Since it doesn't make much sense for a user to log in twice, this view should check if there is a logged in user, and redirect to the post-login view if there is. (See #2882 for which view this should be).
---
**Good First Issue**: This issue is good for first time contributors. If there is not a corresponding pull request for this issue, it is up for grabs. For directions for getting set up, see our [Getting Started Guide](https://warehouse.pypa.io/development/getting-started/). If you are working on this issue and have questions, please feel free to ask them here, [`#pypa-dev` on Freenode](https://webchat.freenode.net/?channels=%23pypa-dev), or the [pypa-dev mailing list](https://groups.google.com/forum/#!forum/pypa-dev).
| @waseem18 Would you like to take this on next?
(Until he says yes, this is still up for grabs.)
Absolutely Yes!
I'll start looking into it right away :+1:
Cool, thanks, @waseem18 -- if you jump into IRC I have a few more suggestions for you.
Sure, Hopping in
This issue also exists for the view `accounts.register` | 2018-02-19T18:15:51Z | [] | [] |
pypi/warehouse | 2,988 | pypi__warehouse-2988 | [
"2802"
] | f9acc7c8cea658618d6c8b4ce306eb25dc87a2a9 | diff --git a/warehouse/accounts/views.py b/warehouse/accounts/views.py
--- a/warehouse/accounts/views.py
+++ b/warehouse/accounts/views.py
@@ -263,6 +263,9 @@ def register(request, _form_class=RegistrationForm):
require_methods=False,
)
def request_password_reset(request, _form_class=RequestPasswordResetForm):
+ if request.authenticated_userid is not None:
+ return HTTPSeeOther(request.route_path('index'))
+
user_service = request.find_service(IUserService, context=None)
form = _form_class(request.POST, user_service=user_service)
@@ -288,6 +291,9 @@ def request_password_reset(request, _form_class=RequestPasswordResetForm):
require_methods=False,
)
def reset_password(request, _form_class=ResetPasswordForm):
+ if request.authenticated_userid is not None:
+ return HTTPSeeOther(request.route_path('index'))
+
user_service = request.find_service(IUserService, context=None)
token_service = request.find_service(ITokenService, name="password")
| diff --git a/tests/unit/accounts/test_views.py b/tests/unit/accounts/test_views.py
--- a/tests/unit/accounts/test_views.py
+++ b/tests/unit/accounts/test_views.py
@@ -581,6 +581,15 @@ def test_request_password_reset_with_wrong_credentials(
]
assert send_password_reset_email.calls == []
+ def test_redirect_authenticated_user(self):
+ pyramid_request = pretend.stub(authenticated_userid=1)
+ pyramid_request.route_path = pretend.call_recorder(
+ lambda a: '/the-redirect'
+ )
+ result = views.request_password_reset(pyramid_request)
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/the-redirect"
+
class TestResetPassword:
@@ -872,6 +881,15 @@ def test_reset_password_password_date_changed(self, pyramid_request):
),
]
+ def test_redirect_authenticated_user(self):
+ pyramid_request = pretend.stub(authenticated_userid=1)
+ pyramid_request.route_path = pretend.call_recorder(
+ lambda a: '/the-redirect'
+ )
+ result = views.reset_password(pyramid_request)
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/the-redirect"
+
class TestVerifyEmail:
| Redirect from password reset pages if user is logged in
Currently the two password reset views will still render even if there's a user logged in:
* `accounts.request-password-reset`
* `accounts.reset-password`
Since it doesn't make much sense for a logged in user to request a password reset, or reset their password, these views should check if there is a logged in user, and redirect to the `index` route if there is.
---
**Good First Issue**: This issue is good for first time contributors. If there is not a corresponding pull request for this issue, it is up for grabs. For directions for getting set up, see our [Getting Started Guide](https://warehouse.pypa.io/development/getting-started/). If you are working on this issue and have questions, please feel free to ask them here, [`#pypa-dev` on Freenode](https://webchat.freenode.net/?channels=%23pypa-dev), or the [pypa-dev mailing list](https://groups.google.com/forum/#!forum/pypa-dev).
| I am a beginner and would like to work on this issue. Can i work on it and be guided along?
@ShubhGupta2125 Absolutely!
Take a look at our [Getting Started Guide](https://warehouse.readthedocs.io/development/getting-started/) for setting up your development environment and feel free to ask any questions here.
@ShubhGupta2125 - are you still on this? Otherwise I would like to tackle it as a first to get involved. (cc: @di) | 2018-02-19T23:06:14Z | [] | [] |
pypi/warehouse | 2,997 | pypi__warehouse-2997 | [
"2701"
] | a37143acf816c50a55e8977ac2da560270fbcf6e | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -1027,7 +1027,13 @@ def file_upload(request):
if is_duplicate:
return Response()
elif is_duplicate is not None:
- raise _exc_with_message(HTTPBadRequest, "File already exists.")
+ raise _exc_with_message(
+ HTTPBadRequest, "File already exists. "
+ "See " +
+ request.route_url(
+ 'help', _anchor='file-name-reuse'
+ )
+ )
# Check to see if the file that was uploaded exists in our filename log
if (request.db.query(
@@ -1037,7 +1043,10 @@ def file_upload(request):
raise _exc_with_message(
HTTPBadRequest,
"This filename has previously been used, you should use a "
- "different version.",
+ "different version. "
+ "See " + request.route_url(
+ 'help', _anchor='file-name-reuse'
+ ),
)
# Check to see if uploading this file would create a duplicate sdist
| diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -1615,16 +1615,23 @@ def test_upload_fails_with_previously_used_filename(self, pyramid_config,
})
db_request.db.add(Filename(filename=filename))
+ db_request.route_url = pretend.call_recorder(
+ lambda route, **kw: "/the/help/url/"
+ )
with pytest.raises(HTTPBadRequest) as excinfo:
legacy.file_upload(db_request)
resp = excinfo.value
+ assert db_request.route_url.calls == [
+ pretend.call('help', _anchor='file-name-reuse')
+ ]
assert resp.status_code == 400
assert resp.status == (
"400 This filename has previously been used, you should use a "
- "different version."
+ "different version. "
+ "See /the/help/url/"
)
def test_upload_noop_with_existing_filename_same_content(self,
@@ -1722,14 +1729,22 @@ def test_upload_fails_with_existing_filename_diff_content(self,
),
),
)
-
+ db_request.route_url = pretend.call_recorder(
+ lambda route, **kw: "/the/help/url/"
+ )
with pytest.raises(HTTPBadRequest) as excinfo:
legacy.file_upload(db_request)
resp = excinfo.value
+ assert db_request.route_url.calls == [
+ pretend.call('help', _anchor='file-name-reuse')
+ ]
assert resp.status_code == 400
- assert resp.status == "400 File already exists."
+ assert resp.status == (
+ "400 File already exists. "
+ "See /the/help/url/"
+ )
def test_upload_fails_with_wrong_filename(self, pyramid_config,
db_request):
| "File already exists." Error could use help links
#2670 brings to light that our error when uploading a filename which has already been used, but no longer exists is unclear.
```
HTTPError: 400 Client Error: File already exists. for url: https://upload.pypi.org/legacy/
```
Here are the two scenarios and messaging I believe would be more helpful. We'd need to create the help entry for `https://pypi.org/help/#file-name-reuse` as well.
- Filename has been used, file exists:
```HTTPError: 400 Client Error: File already exists, see https://pypi.org/help/#file-name-reuse. for url: https://upload.pypi.org/legacy/```
- Filename has been used, file no longer exists:
```HTTPError: 400 Client Error: Filename cannot be reused, see https://pypi.org/help/#file-name-reuse. for url: https://upload.pypi.org/legacy/```
| 2018-02-20T19:58:15Z | [] | [] |
|
pypi/warehouse | 3,049 | pypi__warehouse-3049 | [
"2491"
] | f75cc0c56c440c2a516f85edfa16248813f83f9f | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -675,21 +675,24 @@ def file_upload(request):
if request.POST.get("protocol_version", "1") != "1":
raise _exc_with_message(HTTPBadRequest, "Unknown protocol version.")
+ # Check if any fields were supplied as a tuple and have become a
+ # FieldStorage. The 'content' field _should_ be a FieldStorage, however.
+ # ref: https://github.com/pypa/warehouse/issues/2185
+ # ref: https://github.com/pypa/warehouse/issues/2491
+ for field in set(request.POST) - {'content'}:
+ values = request.POST.getall(field)
+ if any(isinstance(value, FieldStorage) for value in values):
+ raise _exc_with_message(
+ HTTPBadRequest,
+ f"{field}: Should not be a tuple.",
+ )
+
# Look up all of the valid classifiers
all_classifiers = request.db.query(Classifier).all()
# Validate and process the incoming metadata.
form = MetadataForm(request.POST)
- # Check if the classifiers were supplied as a tuple
- # ref: https://github.com/pypa/warehouse/issues/2185
- classifiers = request.POST.getall('classifiers')
- if any(isinstance(classifier, FieldStorage) for classifier in classifiers):
- raise _exc_with_message(
- HTTPBadRequest,
- "classifiers: Must be a list, not tuple.",
- )
-
form.classifiers.choices = [
(c.classifier, c.classifier) for c in all_classifiers
]
| diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -839,7 +839,19 @@ def test_fails_invalid_version(self, pyramid_config, pyramid_request,
"filetype": "sdist",
"classifiers": FieldStorage(),
},
- "classifiers: Must be a list, not tuple.",
+ "classifiers: Should not be a tuple.",
+ ),
+
+ # keywords are a FieldStorage
+ (
+ {
+ "metadata_version": "1.2",
+ "name": "example",
+ "version": "1.0",
+ "filetype": "sdist",
+ "keywords": FieldStorage(),
+ },
+ "keywords: Should not be a tuple.",
),
],
)
| Periodically get a cgi.FieldStorage instead of a string on uploads
For some reason, and I'm not sure why it happens, sometimes the POST dictionary contains cgi.FieldStorage classes instead of strings (I think). We should figure out what cases this happens in, and guard against them however we can.
---
https://sentry.io/python-software-foundation/warehouse-production/issues/187224559/
```
ProgrammingError: can't adapt type 'cgi_FieldStorage'
File "sqlalchemy/engine/base.py", line 1182, in _execute_context
context)
File "sqlalchemy/engine/default.py", line 470, in do_execute
cursor.execute(statement, parameters)
ProgrammingError: (raised as a result of Query-invoked autoflush; consider using a session.no_autoflush block if this flush is occurring prematurely) (psycopg2.ProgrammingError) can't adapt type 'cgi_FieldStorage' [SQL: 'INSERT INTO releases (name, version, author, author_email, maintainer, maintainer_email, home_page, license, summary, description, keywords, platform, download_url, _pypi_ordering, _pypi_hidden, cheesecake_installability_id, cheesecake_documentation_id, cheesecake_code_kwalitee_id, requires_python, description_from_readme) VALUES (%(name)s, %(version)s, %(author)s, %(author_email)s, %(maintainer)s, %(maintainer_email)s, %(home_page)s, %(license)s, %(summary)s, %(description)s, %(keywords)s, %(platform)s, %(download_url)s, %(_pypi_ordering)s, %(_pypi_hidden)s, %(cheesecake_installability_id)s, %(cheesecake_documentation_id)s, %(cheesecake_code_kwalitee_id)s, %(requires_python)s, %(description_from_readme)s)'] [parameters: {'name': 'mdsplus', 'version': '7.7.6', 'author': 'Tom Fredian,Josh Stillerman,Gabriele Manduchi', 'author_email': '[email protected]', 'maintainer': '', 'maintainer_email': '', 'home_page': 'http://www.mdsplus.org/', 'license': '', 'summary': 'MDSplus Python Objects', 'description': '\n This module provides all of the functionality of MDSplus TDI natively in python.\n All of the MDSplus data types such as signal are represented as python classes.\n ', 'keywords': FieldStorage('keywords', 'physics'), 'platform': '', 'download_url': 'http://www.mdsplus.org/mdsplus_download/python', '_pypi_ordering': None, '_pypi_hidden': False, 'cheesecake_installability_id': None, 'cheesecake_documentation_id': None, 'cheesecake_code_kwalitee_id': None, 'requires_python': '', 'description_from_readme': None}]
(51 additional frame(s) were not displayed)
...
File "warehouse/raven.py", line 41, in raven_tween
return handler(request)
File "warehouse/cache/http.py", line 69, in conditional_http_tween
response = handler(request)
File "warehouse/cache/http.py", line 33, in wrapped
return view(context, request)
File "warehouse/csrf.py", line 38, in wrapped
return view(context, request)
File "warehouse/forklift/legacy.py", line 791, in file_upload
.filter(Release.project == project)
ProgrammingError: (raised as a result of Query-invoked autoflush; consider using a session.no_autoflush block if this flush is occurring prematurely) (psycopg2.ProgrammingError) can't adapt type 'cgi_FieldStorage' [SQL: 'INSERT INTO releases (name, version, author, author_email, maintainer, maintainer_email, home_page, license, summary, description, keywords, platform, download_url, _pypi_ordering, _pypi_hidden, cheesecake_installability_id, cheesecake_documentation_id, cheesecake_code_kwalitee_id, requires_python, description_from_readme) VALUES (%(name)s, %(version)s, %(author)s, %(author_email)s, %(maintainer)s, %(maintainer_email)s, %(home_page)s, %(license)s, %(summary)s, %(description)s, %(keywords)s, %(platform)s, %(download_url)s, %(_pypi_ordering)s, %(_pypi_hidden)s, %(cheesecake_installability_id)s, %(cheesecake_documentation_id)s, %(cheesecake_code_kwalitee_id)s, %(requires_python)s, %(description_from_readme)s)'] [parameters: {'name': 'mdsplus', 'version': '7.7.6', 'author': 'Tom Fredian,Josh Stillerman,Gabriele Manduchi', 'author_email': '[email protected]', 'maintainer': '', 'maintainer_email': '', 'home_page': 'http://www.mdsplus.org/', 'license': '', 'summary': 'MDSplus Python Objects', 'description': '\n This module provides all of the functionality of MDSplus TDI natively in python.\n All of the MDSplus data types such as signal are represented as python classes.\n ', 'keywords': FieldStorage('keywords', 'physics'), 'platform': '', 'download_url': 'http://www.mdsplus.org/mdsplus_download/python', '_pypi_ordering': None, '_pypi_hidden': False, 'cheesecake_installability_id': None, 'cheesecake_documentation_id': None, 'cheesecake_code_kwalitee_id': None, 'requires_python': '', 'description_from_readme': None}]
```
| Quick attempt at debugging this, according to the Sentry traceback the error happens when trying to insert a new `Release` including `'keywords': FieldStorage('keywords', 'physics')` as a parameter for the query.
I believe the sequence would be:
1. a POST request is made
2. a WSGI environment is created and parsed by Webob
3. a Webob `Request` object contains a `Multidict` in its POST attribute from the parsed body of the request which somehow causes keywords to be a `FieldStorage` instance
4. The view [constructs a MetadataForm from request.POST](https://github.com/pypa/warehouse/blob/master/warehouse/forklift/legacy.py#L630) and successfully validates the form including the FieldStorage as a StringField.
5. The view then [constructs and adds a new `Release`](https://github.com/pypa/warehouse/blob/master/warehouse/forklift/legacy.py#L738) which causes the error.
Would it be possible to check the body of the request?
Seems like we could do something similar to #2655 here. | 2018-02-23T22:03:20Z | [] | [] |
pypi/warehouse | 3,056 | pypi__warehouse-3056 | [
"2884"
] | 8a82f22d8df3661940f78d599bf58f28cee9eac7 | diff --git a/warehouse/utils/project.py b/warehouse/utils/project.py
--- a/warehouse/utils/project.py
+++ b/warehouse/utils/project.py
@@ -19,7 +19,7 @@
def confirm_project(project, request, fail_route):
- confirm = request.POST.get("confirm")
+ confirm = request.POST.get("confirm_project_name")
project_name = project.normalized_name
if not confirm:
request.session.flash(
| diff --git a/tests/unit/admin/views/test_projects.py b/tests/unit/admin/views/test_projects.py
--- a/tests/unit/admin/views/test_projects.py
+++ b/tests/unit/admin/views/test_projects.py
@@ -434,7 +434,7 @@ def test_no_confirm(self):
def test_wrong_confirm(self):
project = pretend.stub(normalized_name='foo')
request = pretend.stub(
- POST={"confirm": "bar"},
+ POST={"confirm_project_name": "bar"},
session=pretend.stub(
flash=pretend.call_recorder(lambda *a, **kw: None),
),
@@ -461,7 +461,7 @@ def test_deletes_project(self, db_request):
db_request.session = pretend.stub(
flash=pretend.call_recorder(lambda *a, **kw: None),
)
- db_request.POST["confirm"] = project.normalized_name
+ db_request.POST["confirm_project_name"] = project.normalized_name
db_request.user = UserFactory.create()
db_request.remote_addr = "192.168.1.1"
diff --git a/tests/unit/manage/test_views.py b/tests/unit/manage/test_views.py
--- a/tests/unit/manage/test_views.py
+++ b/tests/unit/manage/test_views.py
@@ -773,7 +773,7 @@ def test_delete_project_no_confirm(self):
def test_delete_project_wrong_confirm(self):
project = pretend.stub(normalized_name='foo')
request = pretend.stub(
- POST={"confirm": "bar"},
+ POST={"confirm_project_name": "bar"},
session=pretend.stub(
flash=pretend.call_recorder(lambda *a, **kw: None),
),
@@ -801,7 +801,7 @@ def test_delete_project(self, db_request):
db_request.session = pretend.stub(
flash=pretend.call_recorder(lambda *a, **kw: None),
)
- db_request.POST["confirm"] = project.normalized_name
+ db_request.POST["confirm_project_name"] = project.normalized_name
db_request.user = UserFactory.create()
db_request.remote_addr = "192.168.1.1"
diff --git a/tests/unit/utils/test_project.py b/tests/unit/utils/test_project.py
--- a/tests/unit/utils/test_project.py
+++ b/tests/unit/utils/test_project.py
@@ -29,7 +29,7 @@
def test_confirm():
project = stub(normalized_name='foobar')
request = stub(
- POST={'confirm': 'foobar'},
+ POST={'confirm_project_name': 'foobar'},
route_path=call_recorder(lambda *a, **kw: stub()),
session=stub(flash=call_recorder(lambda *a, **kw: stub())),
)
@@ -43,7 +43,7 @@ def test_confirm():
def test_confirm_no_input():
project = stub(normalized_name='foobar')
request = stub(
- POST={'confirm': ''},
+ POST={'confirm_project_name': ''},
route_path=call_recorder(lambda *a, **kw: '/the-redirect'),
session=stub(flash=call_recorder(lambda *a, **kw: stub())),
)
@@ -63,7 +63,7 @@ def test_confirm_no_input():
def test_confirm_incorrect_input():
project = stub(normalized_name='foobar')
request = stub(
- POST={'confirm': 'bizbaz'},
+ POST={'confirm_project_name': 'bizbaz'},
route_path=call_recorder(lambda *a, **kw: '/the-redirect'),
session=stub(flash=call_recorder(lambda *a, **kw: stub())),
)
| Disable 'delete confirm' button until confirmation word is correct
We currently have a modal on `warehouse/templates/manage/settings.html`, that allows the user to confirm that they want to delete their project:
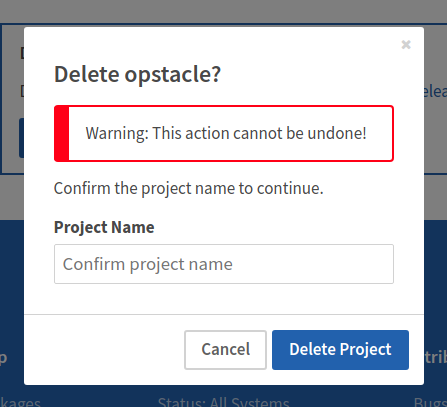
The user is required to enter the project name as an extra security measure. If they get it wrong, we show them this error:

## Proposal
It would be really nice if we could `disable` the delete button until the correct project name is given, e.g.
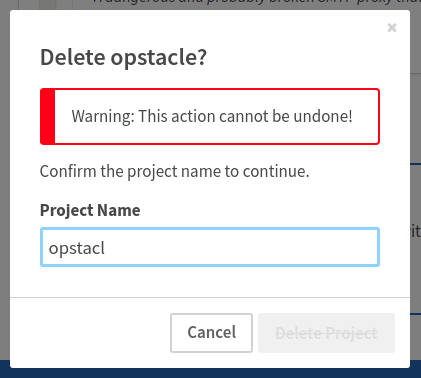
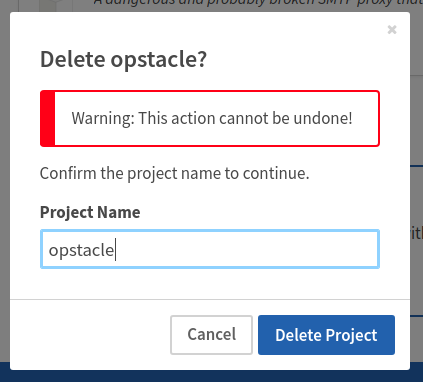
## Notes
We will have several other delete confirmation modals on other pages, sometimes with multiple modals on a single page (e.g. delete release, delete file) - so the code will need to be written to take this into account.
| **Good First Issue**: This issue is good for first time contributors. If there is not a corresponding pull request for this issue, it is up for grabs. For directions for getting set up, see our [Getting Started Guide](https://warehouse.pypa.io/development/getting-started/). If you are working on this issue and have questions, please feel free to ask them here, [`#pypa-dev` on Freenode](https://webchat.freenode.net/?channels=%23pypa-dev), or the [pypa-dev mailing list](https://groups.google.com/forum/#!forum/pypa-dev).
If you're interested in working on this, speak up here, and mention `@di` -- he has some thoughts on adding some light JavaScript touches to broadly handle this and similar instances of this elsewhere in our codebase (as Nicole mentioned in her "Notes" section above). | 2018-02-24T07:24:42Z | [] | [] |
pypi/warehouse | 3,061 | pypi__warehouse-3061 | [
"3059"
] | 81cd1e1eb5db70624909999ee3fe9e8eb328b5f8 | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -676,10 +676,11 @@ def file_upload(request):
raise _exc_with_message(HTTPBadRequest, "Unknown protocol version.")
# Check if any fields were supplied as a tuple and have become a
- # FieldStorage. The 'content' field _should_ be a FieldStorage, however.
+ # FieldStorage. The 'content' and 'gpg_signature' fields _should_ be a
+ # FieldStorage, however.
# ref: https://github.com/pypa/warehouse/issues/2185
# ref: https://github.com/pypa/warehouse/issues/2491
- for field in set(request.POST) - {'content'}:
+ for field in set(request.POST) - {'content', 'gpg_signature'}:
values = request.POST.getall(field)
if any(isinstance(value, FieldStorage) for value in values):
raise _exc_with_message(
| diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -1066,17 +1066,19 @@ def test_successful_upload(self, tmpdir, monkeypatch, pyramid_config,
db_request.user = user
db_request.remote_addr = "10.10.10.40"
+
+ content = FieldStorage()
+ content.filename = filename
+ content.file = io.BytesIO(b"A fake file.")
+ content.type = "application/tar"
+
db_request.POST = MultiDict({
"metadata_version": "1.2",
"name": project.name,
"version": release.version,
"filetype": "sdist",
"pyversion": "source",
- "content": pretend.stub(
- filename=filename,
- file=io.BytesIO(b"A fake file."),
- type="application/tar",
- ),
+ "content": content,
})
db_request.POST.extend([
("classifiers", "Environment :: Other Environment"),
@@ -1084,13 +1086,14 @@ def test_successful_upload(self, tmpdir, monkeypatch, pyramid_config,
db_request.POST.update(digests)
if has_signature:
- db_request.POST["gpg_signature"] = pretend.stub(
- filename=filename + ".asc",
- file=io.BytesIO(
- b"-----BEGIN PGP SIGNATURE-----\n"
- b" This is a Fake Signature"
- ),
+ gpg_signature = FieldStorage()
+ gpg_signature.filename = filename + ".asc"
+ gpg_signature.file = io.BytesIO(
+ b"-----BEGIN PGP SIGNATURE-----\n"
+ b" This is a Fake Signature"
)
+ db_request.POST["gpg_signature"] = gpg_signature
+ assert isinstance(db_request.POST["gpg_signature"], FieldStorage)
@pretend.call_recorder
def storage_service_store(path, file_path, *, meta):
| gpg_signature: Should not be a tuple
Sometime in the last two weeks, gpg-signed uploads to https://upload.pypi.org/legacy/ began to break with the following error message:
```
Uploading distributions to https://upload.pypi.org/legacy/
Signing ${snip}.whl
You need a passphrase to unlock the secret key for
user: "${snip}"
2048-bit RSA key, ID ${snip}, created ${snip}
Uploading ${snip}.whl
HTTPError: 400 Client Error: gpg_signature: Should not be a tuple. for url: https://upload.pypi.org/legacy/
```
This seems potentially related to #3049 ?
This is in a venv using twine for the upload and the following dependency versions.
```
$ twine-deps.venv/bin/twine --version
twine version 1.9.1 (pkginfo: 1.4.1, requests: 2.18.4, setuptools: 38.5.1,
requests-toolbelt: 0.8.0, tqdm: 4.19.5)
$ twine-deps.venv/bin/python --version
Python 2.7.14
```
Neither our invoke of `twine`, nor the dependencies have changed in the last two weeks.
| 2018-02-24T20:23:00Z | [] | [] |
|
pypi/warehouse | 3,111 | pypi__warehouse-3111 | [
"2850"
] | 0e5a3248cb147c7ce6f7937ccdd954f937ed7d1b | diff --git a/warehouse/packaging/search.py b/warehouse/packaging/search.py
--- a/warehouse/packaging/search.py
+++ b/warehouse/packaging/search.py
@@ -34,7 +34,7 @@
class Project(DocType):
name = Text()
- normalized_name = Text(analyzer=NameAnalyzer, index_options="docs")
+ normalized_name = Text(analyzer=NameAnalyzer)
version = Keyword(multi=True)
latest_version = Keyword()
summary = Text(analyzer="snowball")
diff --git a/warehouse/views.py b/warehouse/views.py
--- a/warehouse/views.py
+++ b/warehouse/views.py
@@ -11,6 +11,7 @@
# limitations under the License.
import collections
+import re
from pyramid.httpexceptions import (
HTTPException, HTTPSeeOther, HTTPMovedPermanently, HTTPNotFound,
@@ -248,20 +249,13 @@ def classifiers(request):
def search(request):
q = request.params.get("q", '')
+ q = q.replace("'", '"')
if q:
- should = []
- for field in SEARCH_FIELDS:
- kw = {"query": q}
- if field in SEARCH_BOOSTS:
- kw["boost"] = SEARCH_BOOSTS[field]
- should.append(Q("match", **{field: kw}))
-
- # Add a prefix query if ``q`` is longer than one character.
- if len(q) > 1:
- should.append(Q('prefix', normalized_name=q))
-
- query = request.es.query("dis_max", queries=should)
+ bool_query = gather_es_queries(q)
+
+ query = request.es.query(bool_query)
+
query = query.suggest("name_suggestion", q, term={"field": "name"})
else:
query = request.es.query()
@@ -372,3 +366,45 @@ def force_status(request):
raise exception_response(int(request.matchdict["status"]))
except KeyError:
raise exception_response(404) from None
+
+
+def filter_query(s):
+ """
+ Filters given query with the below regex
+ and returns lists of quoted and unquoted strings
+ """
+ matches = re.findall(r'(?:"([^"]*)")|([^"]*)', s)
+ result_quoted = [t[0].strip() for t in matches if t[0]]
+ result_unquoted = [t[1].strip() for t in matches if t[1]]
+ return result_quoted, result_unquoted
+
+
+def form_query(query_type, query):
+ """
+ Returns a multi match query
+ """
+ fields = [
+ field + "^" + str(SEARCH_BOOSTS[field])
+ if field in SEARCH_BOOSTS else field
+ for field in SEARCH_FIELDS
+ ]
+ return Q('multi_match', fields=fields,
+ query=query, type=query_type
+ )
+
+
+def gather_es_queries(q):
+ quoted_string, unquoted_string = filter_query(q)
+ must = [
+ form_query("phrase", i) for i in quoted_string
+ ] + [
+ form_query("best_fields", i) for i in unquoted_string
+ ]
+
+ bool_query = Q('bool', must=must)
+
+ # Allow to optionally match on prefix
+ # if ``q`` is longer than one character.
+ if len(q) > 1:
+ bool_query = bool_query | Q("prefix", normalized_name=q)
+ return bool_query
| diff --git a/tests/unit/test_views.py b/tests/unit/test_views.py
--- a/tests/unit/test_views.py
+++ b/tests/unit/test_views.py
@@ -14,7 +14,6 @@
import pretend
import pytest
-from elasticsearch_dsl import Q
from webob.multidict import MultiDict
from pyramid.httpexceptions import (
@@ -23,9 +22,9 @@
from warehouse import views
from warehouse.views import (
- SEARCH_BOOSTS, SEARCH_FIELDS, classifiers, current_user_indicator,
- forbidden, health, httpexception_view, index, robotstxt, opensearchxml,
- search, force_status, flash_messages, forbidden_include
+ classifiers, current_user_indicator, forbidden, health,
+ httpexception_view, index, robotstxt, opensearchxml, search, force_status,
+ flash_messages, forbidden_include
)
from ..common.db.accounts import UserFactory
@@ -207,17 +206,6 @@ def test_esi_flash_messages():
class TestSearch:
- def _gather_es_queries(self, q):
- queries = []
- for field in SEARCH_FIELDS:
- kw = {"query": q}
- if field in SEARCH_BOOSTS:
- kw["boost"] = SEARCH_BOOSTS[field]
- queries.append(Q("match", **{field: kw}))
- if len(q) > 1:
- queries.append(Q("prefix", normalized_name=q))
- return queries
-
@pytest.mark.parametrize("page", [None, 1, 5])
def test_with_a_query(self, monkeypatch, db_request, page):
params = MultiDict({"q": "foo bar"})
@@ -257,8 +245,59 @@ def test_with_a_query(self, monkeypatch, db_request, page):
assert url_maker_factory.calls == [pretend.call(db_request)]
assert db_request.es.query.calls == [
pretend.call(
- "dis_max",
- queries=self._gather_es_queries(params["q"])
+ views.gather_es_queries(params["q"])
+ )
+ ]
+ assert es_query.suggest.calls == [
+ pretend.call(
+ "name_suggestion",
+ params["q"],
+ term={"field": "name"},
+ ),
+ ]
+
+ @pytest.mark.parametrize("page", [None, 1, 5])
+ def test_with_exact_phrase_query(self, monkeypatch, db_request, page):
+ params = MultiDict({"q": '"foo bar"'})
+ if page is not None:
+ params["page"] = page
+ db_request.params = params
+
+ sort = pretend.stub()
+ suggest = pretend.stub(
+ sort=pretend.call_recorder(lambda *a, **kw: sort),
+ )
+ es_query = pretend.stub(
+ suggest=pretend.call_recorder(lambda *a, **kw: suggest),
+ )
+ db_request.es = pretend.stub(
+ query=pretend.call_recorder(lambda *a, **kw: es_query)
+ )
+
+ page_obj = pretend.stub(page_count=(page or 1) + 10,
+ item_count=(page or 1) + 10
+ )
+ page_cls = pretend.call_recorder(lambda *a, **kw: page_obj)
+ monkeypatch.setattr(views, "ElasticsearchPage", page_cls)
+
+ url_maker = pretend.stub()
+ url_maker_factory = pretend.call_recorder(lambda request: url_maker)
+ monkeypatch.setattr(views, "paginate_url_factory", url_maker_factory)
+
+ assert search(db_request) == {
+ "page": page_obj,
+ "term": params.get("q", ''),
+ "order": params.get("o", ''),
+ "applied_filters": [],
+ "available_filters": [],
+ }
+ assert page_cls.calls == [
+ pretend.call(suggest, url_maker=url_maker, page=page or 1),
+ ]
+ assert url_maker_factory.calls == [pretend.call(db_request)]
+ assert db_request.es.query.calls == [
+ pretend.call(
+ views.gather_es_queries(params["q"])
)
]
assert es_query.suggest.calls == [
@@ -308,8 +347,7 @@ def test_with_a_single_char_query(self, monkeypatch, db_request, page):
assert url_maker_factory.calls == [pretend.call(db_request)]
assert db_request.es.query.calls == [
pretend.call(
- "dis_max",
- queries=self._gather_es_queries(params["q"])
+ views.gather_es_queries(params["q"])
)
]
assert es_query.suggest.calls == [
@@ -380,8 +418,7 @@ def test_with_an_ordering(self, monkeypatch, db_request, page, order,
assert url_maker_factory.calls == [pretend.call(db_request)]
assert db_request.es.query.calls == [
pretend.call(
- "dis_max",
- queries=self._gather_es_queries(params["q"])
+ views.gather_es_queries(params["q"])
)
]
assert es_query.suggest.calls == [
@@ -455,8 +492,7 @@ def test_with_classifiers(self, monkeypatch, db_request, page):
assert url_maker_factory.calls == [pretend.call(db_request)]
assert db_request.es.query.calls == [
pretend.call(
- "dis_max",
- queries=self._gather_es_queries(params["q"])
+ views.gather_es_queries(params["q"])
)
]
assert es_query.suggest.calls == [
| Support searching for an exact phrase
The search box does not appear to have a way to search for a specific exact phrase.
Based on how other search engines work, putting a phrase in quotes should require an exact phrase match.
However, currently searches with and without quotes produce the same result:
https://pypi.org/search/?q=%22Image+processing+routines+for+SciPy%22
and
https://pypi.org/search/?q=Image+processing+routines+for+SciPy
I would have expected the first one with quotes to only produce the result containing the exact phrase.
---
**Good First Issue**: This issue is good for first time contributors. If there is not a corresponding pull request for this issue, it is up for grabs. For directions for getting set up, see our [Getting Started Guide](https://warehouse.pypa.io/development/getting-started/). If you are working on this issue and have questions, please feel free to ask them here, [`#pypa-dev` on Freenode](https://webchat.freenode.net/?channels=%23pypa-dev), or the [pypa-dev mailing list](https://groups.google.com/forum/#!forum/pypa-dev).
| Thanks for your report, @pv, and sorry for the slow response!
The folks working on Warehouse have gotten [funding to concentrate on improving and deploying Warehouse](https://pyfound.blogspot.com/2017/11/the-psf-awarded-moss-grant-pypi.html), and have kicked off work towards [our development roadmap](https://wiki.python.org/psf/WarehouseRoadmap) -- the most urgent task is to improve Warehouse to the point where we can redirect pypi.python.org to pypi.org so the site is more sustainable and reliable.
We discussed this issue [in our meeting today](https://wiki.python.org/psf/PackagingWG/2018-02-12-Warehouse) to prioritize it. Since search in Warehouse is already much better than search on legacy PyPI, but users will probably expect search to work as you suggest, I've moved this issue to a future milestone that we'll work on in the next few months.
Thanks, @pv, and sorry again for the wait.
Note to people thinking about contributing to Warehouse: this would be a great first issue for a new contributor to tackle if the new contributor were already familiar with Elasticsearch.
I'm looking into this issue.
This doesn't look like a simple `match` to `phrase_match` change.
@waseem18 Yeah, I'm not _totally_ sure that this issue is actually a "good first issue" -- elasticsearch tuning has generally been pretty tricky in my experience. But since you're not a new contributor anymore, should be a good issue for you. 🙂
Update:
After changing `match` to `match_phrase` at [this line](https://github.com/pypa/warehouse/blob/598963368bb71ea2310a6b0220327f7d90e63591/warehouse/views.py#L260) and searching Warehouse with string containing spaces (example: cli github), we get `Transport Error`.
`
elasticsearch.exceptions.TransportError: TransportError(500, 'search_phase_execution_exception', 'field "normalized_name" was indexed without position data; cannot run PhraseQuery (phrase=normalized_name:"cli github")')
`
As phrase queries require `index_options: positions`- I changed
`normalized_name = Text(analyzer=NameAnalyzer, index_options="docs")` to `normalized_name = Text(analyzer=NameAnalyzer, index_options="positions")` [here](https://github.com/pypa/warehouse/blob/598963368bb71ea2310a6b0220327f7d90e63591/warehouse/packaging/search.py#L37) and then reindexed.
At this point the search functionality works but I found the results are not efficient.
@waseem18 what do you mean "not efficient"? I would be happy to help fine-tune the queries
@waseem18 Maybe you have done some profiling and you have some specific performance numbers to share?
@HonzaKral By not efficient I mean the search results are way better when `index_options=docs`.
For example when we search `image processing routines`, `index_options=docs` gives good results while `index_options=positions` doesn't give any result.
This is same with queries containing spaces.
@waseem18 it's going to be helpful if you give concrete numbers or results, I think. Saying "good results" might mean that there are a lot of them, or they're relevant, or they're well ordered... feel free to cut and paste or take screenshots to show what you mean. :)
Sure @brainwane
In this particular case there should be no difference between which documents match with various `index_options` as long as the query remains the same. Only ordering (`_score`) of hits would be different where `docs` doesn't take into account frequency (how many times the word occurs in the document). `positions` is the default and should be used here
Thanks for the information @HonzaKral I'll get back with some examples and screenshots.
@HonzaKral Thanks for the help.
- Tweaked `index_options` to `positions` for Package index and respective `match_phrase` change.
- Changed `SEARCH_BOOST` value of `description` from 5 to 10 which I believe improved phrase queries.
- Attached some screenshots which show how relevant the results are without and with double quotes respectively.
1.
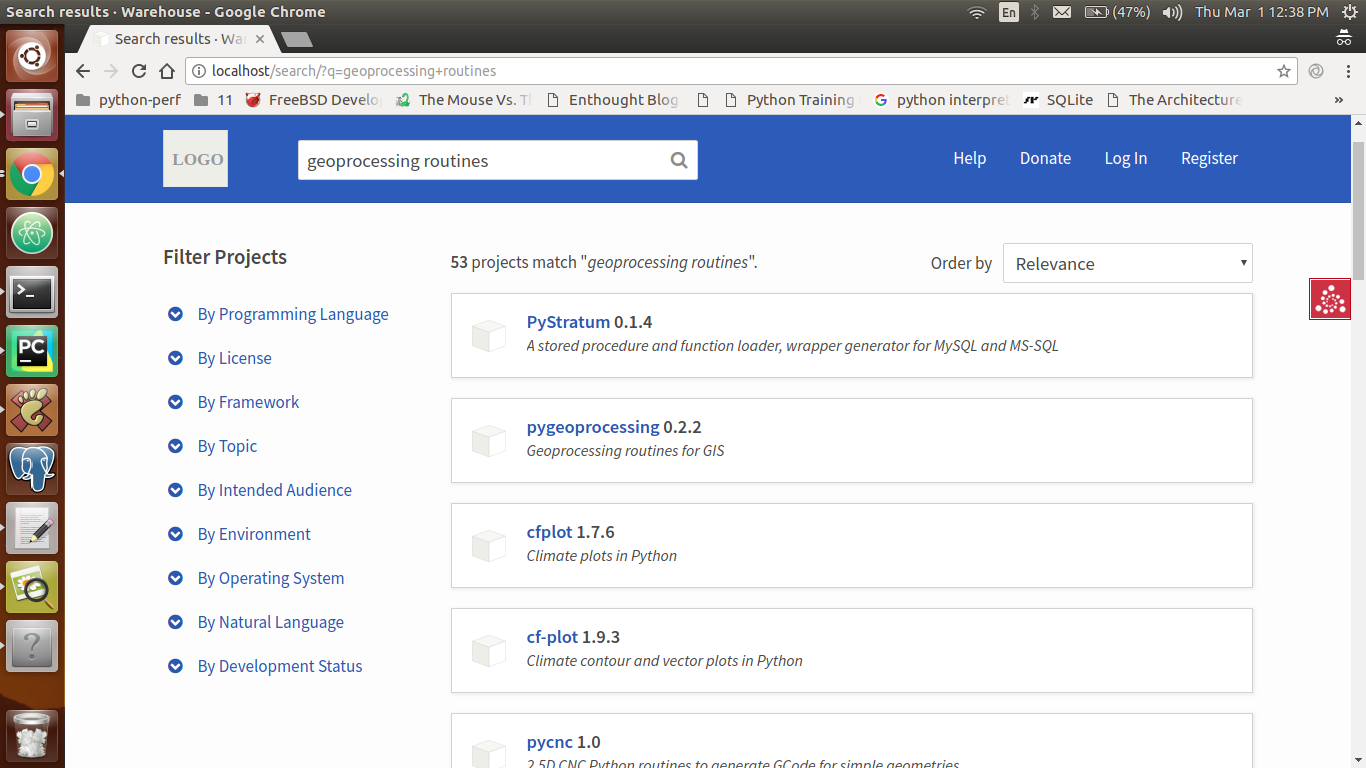
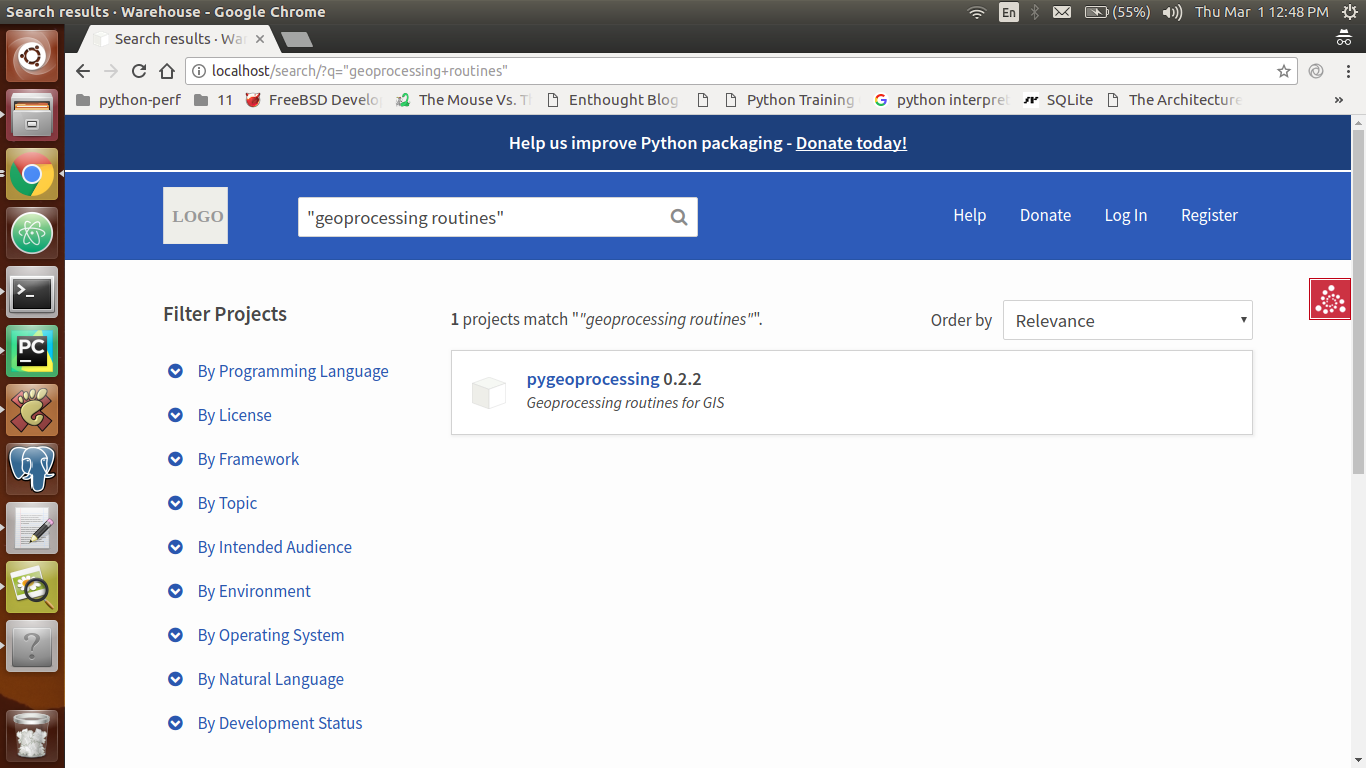
2.
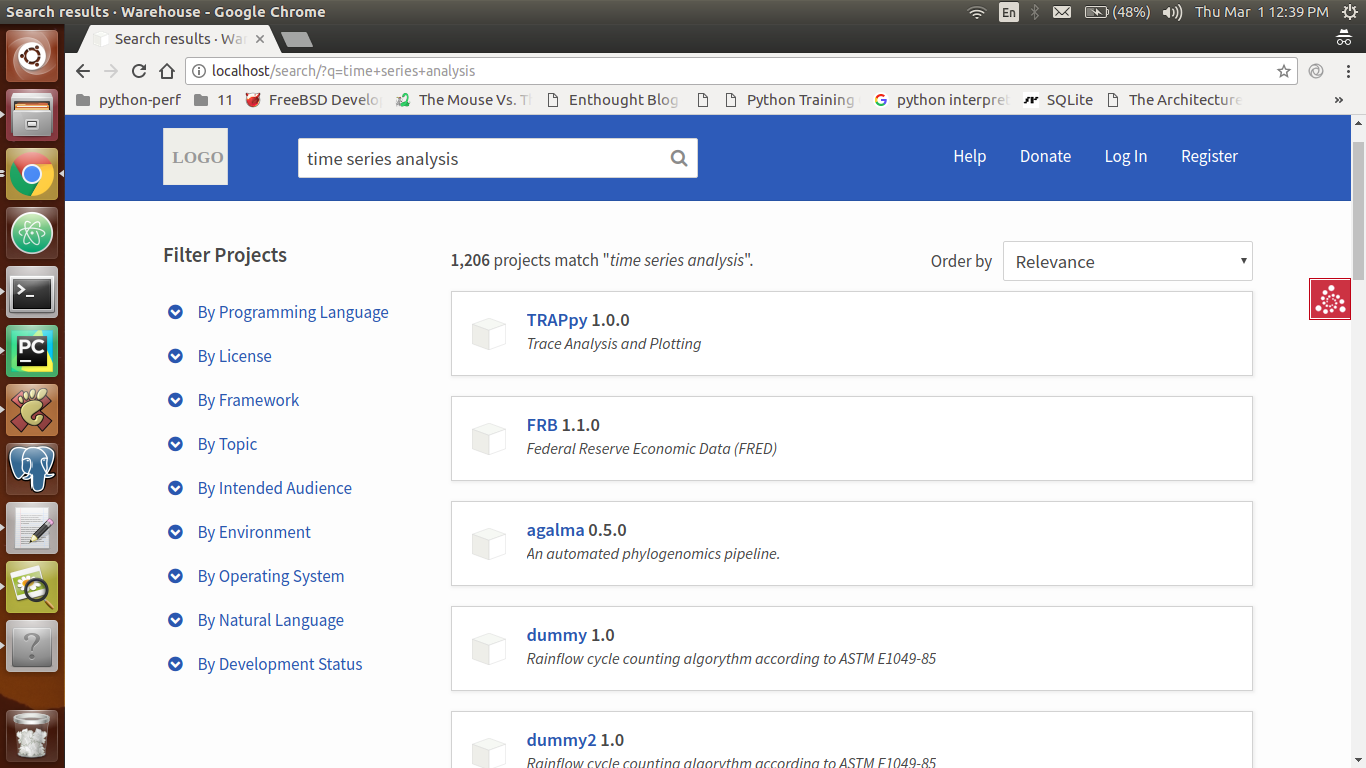
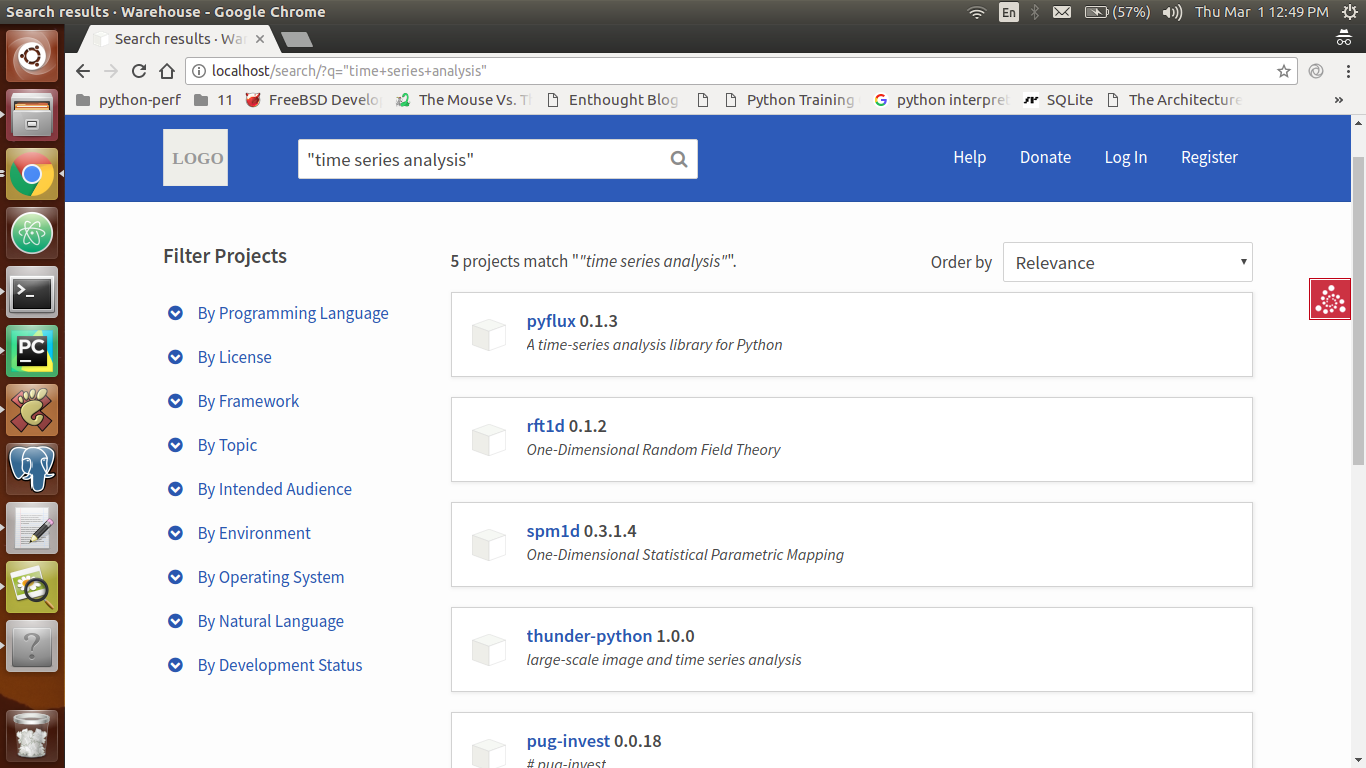
3.
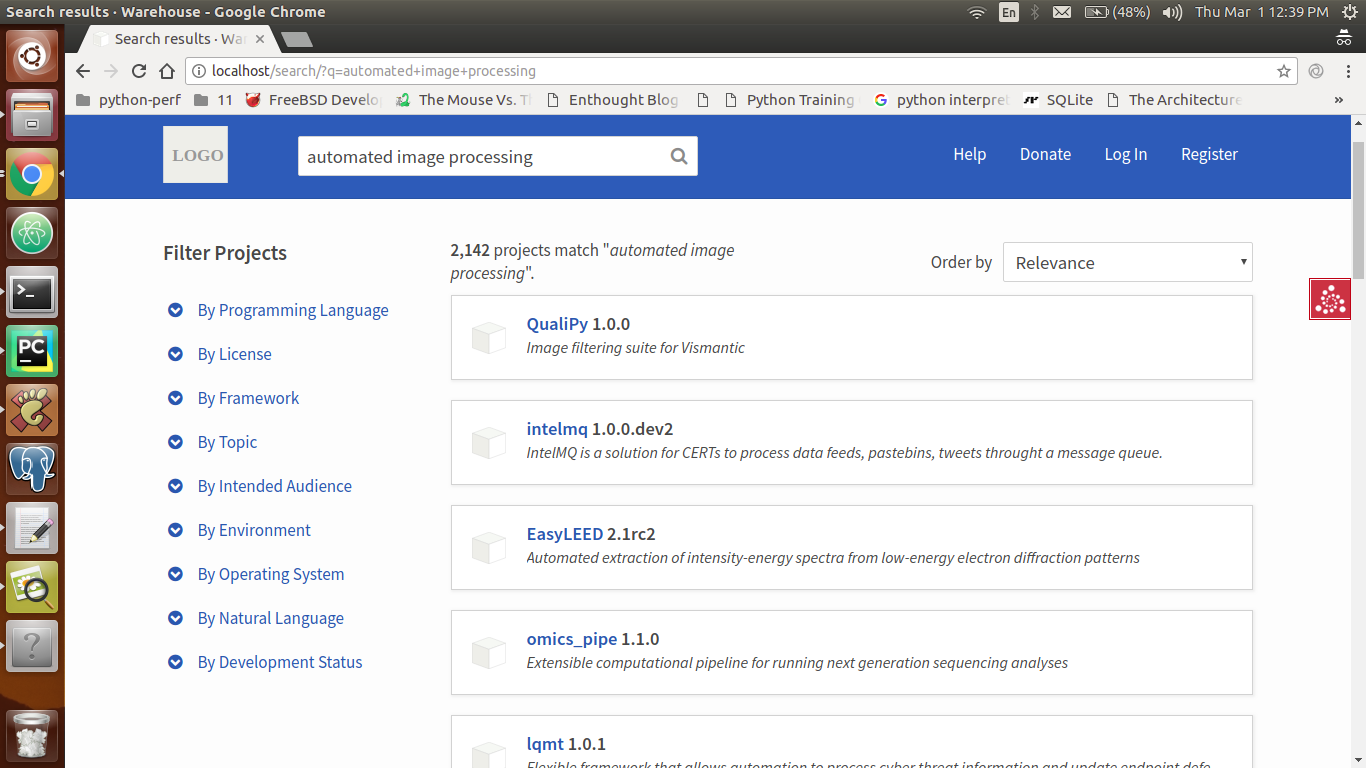
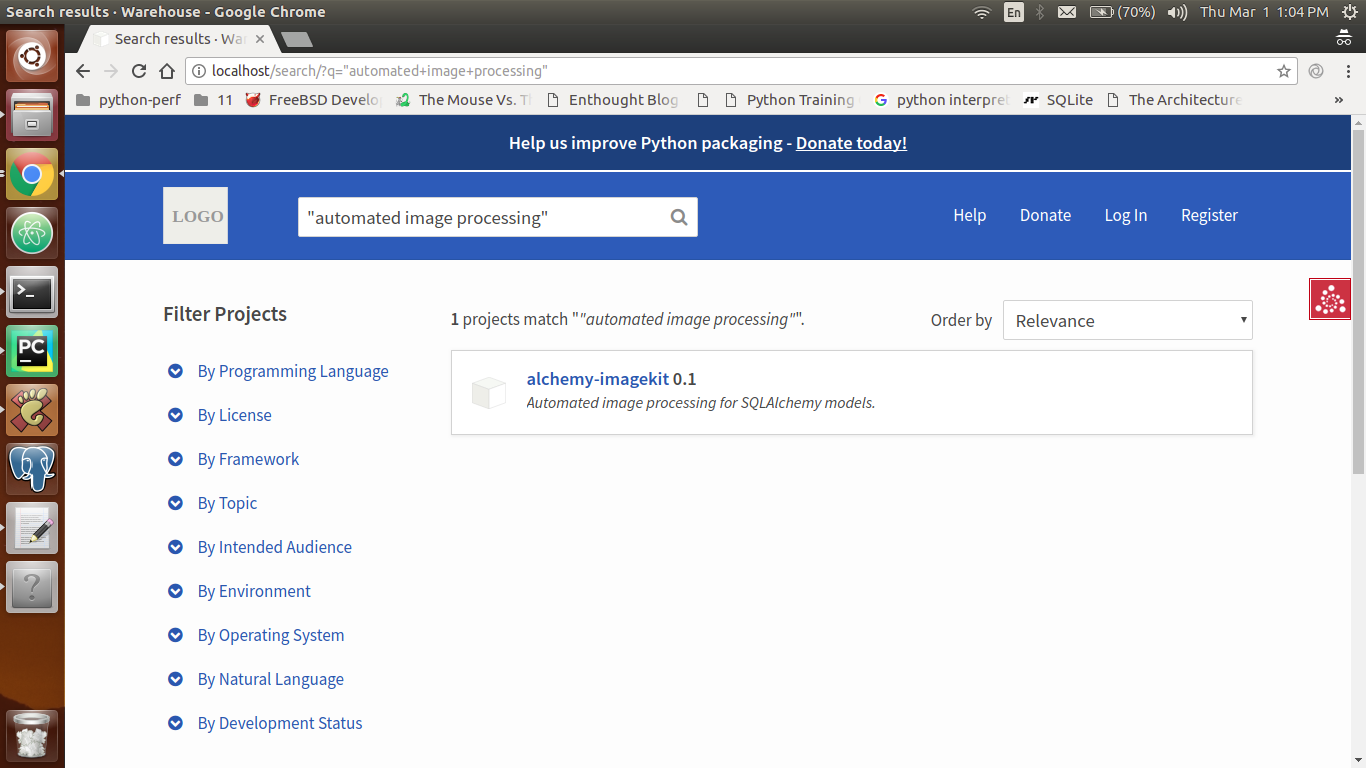
Note that users expect that it's also possible to do queries containing
both exact and inexact matches (probably should be ANDed), along the
lines of:
"image processing" geo
good use case @pv
Will have that in mind | 2018-03-01T15:21:49Z | [] | [] |
pypi/warehouse | 3,113 | pypi__warehouse-3113 | [
"445"
] | eb6c8be420578d7a2247d6d2a736a276eef97a83 | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -835,79 +835,67 @@ def file_upload(request):
)
try:
+ canonical_version = packaging.utils.canonicalize_version(
+ form.version.data
+ )
release = (
request.db.query(Release)
- .filter(
- (Release.project == project) &
- (Release.version == form.version.data)).one()
+ .filter(
+ (Release.project == project) &
+ (Release.canonical_version == canonical_version)
+ )
+ .one()
)
except NoResultFound:
- # We didn't find a release with the exact version string, try and see
- # if one exists with an equivalent parsed version
- releases = (
- request.db.query(Release)
- .filter(Release.project == project)
- .all()
- )
- versions = {
- packaging.version.parse(release.version): release
- for release in releases
- }
-
- try:
- release = versions[packaging.version.parse(form.version.data)]
- except KeyError:
- release = Release(
- project=project,
- _classifiers=[
- c for c in all_classifiers
- if c.classifier in form.classifiers.data
- ],
- _pypi_hidden=False,
- dependencies=list(_construct_dependencies(
- form,
- {
- "requires": DependencyKind.requires,
- "provides": DependencyKind.provides,
- "obsoletes": DependencyKind.obsoletes,
- "requires_dist": DependencyKind.requires_dist,
- "provides_dist": DependencyKind.provides_dist,
- "obsoletes_dist": DependencyKind.obsoletes_dist,
- "requires_external": DependencyKind.requires_external,
- "project_urls": DependencyKind.project_url,
- }
- )),
- canonical_version=packaging.utils.canonicalize_version(
- form.version.data
- ),
- **{
- k: getattr(form, k).data
- for k in {
- # This is a list of all the fields in the form that we
- # should pull off and insert into our new release.
- "version",
- "summary", "description", "license",
- "author", "author_email",
- "maintainer", "maintainer_email",
- "keywords", "platform",
- "home_page", "download_url",
- "requires_python",
- }
+ release = Release(
+ project=project,
+ _classifiers=[
+ c for c in all_classifiers
+ if c.classifier in form.classifiers.data
+ ],
+ _pypi_hidden=False,
+ dependencies=list(_construct_dependencies(
+ form,
+ {
+ "requires": DependencyKind.requires,
+ "provides": DependencyKind.provides,
+ "obsoletes": DependencyKind.obsoletes,
+ "requires_dist": DependencyKind.requires_dist,
+ "provides_dist": DependencyKind.provides_dist,
+ "obsoletes_dist": DependencyKind.obsoletes_dist,
+ "requires_external": DependencyKind.requires_external,
+ "project_urls": DependencyKind.project_url,
}
- )
- request.db.add(release)
- # TODO: This should be handled by some sort of database trigger or
- # a SQLAlchemy hook or the like instead of doing it inline in
- # this view.
- request.db.add(
- JournalEntry(
- name=release.project.name,
- version=release.version,
- action="new release",
- submitted_by=request.user,
- submitted_from=request.remote_addr,
- ),
- )
+ )),
+ canonical_version=canonical_version,
+ **{
+ k: getattr(form, k).data
+ for k in {
+ # This is a list of all the fields in the form that we
+ # should pull off and insert into our new release.
+ "version",
+ "summary", "description", "license",
+ "author", "author_email",
+ "maintainer", "maintainer_email",
+ "keywords", "platform",
+ "home_page", "download_url",
+ "requires_python",
+ }
+ }
+ )
+ request.db.add(release)
+ # TODO: This should be handled by some sort of database trigger or
+ # a SQLAlchemy hook or the like instead of doing it inline in
+ # this view.
+ request.db.add(
+ JournalEntry(
+ name=release.project.name,
+ version=release.version,
+ action="new release",
+ submitted_by=request.user,
+ submitted_from=request.remote_addr,
+ ),
+ )
# TODO: We need a better solution to this than to just do it inline inside
# this method. Ideally the version field would just be sortable, but
diff --git a/warehouse/packaging/models.py b/warehouse/packaging/models.py
--- a/warehouse/packaging/models.py
+++ b/warehouse/packaging/models.py
@@ -14,6 +14,7 @@
from collections import OrderedDict
+import packaging.utils
from citext import CIText
from pyramid.security import Allow
from pyramid.threadlocal import get_current_request
@@ -133,13 +134,16 @@ class Project(SitemapMixin, db.ModelBase):
def __getitem__(self, version):
session = orm.object_session(self)
+ canonical_version = packaging.utils.canonicalize_version(version)
try:
return (
session.query(Release)
- .filter((Release.project == self) &
- (Release.version == version))
- .one()
+ .filter(
+ (Release.project == self) &
+ (Release.canonical_version == canonical_version)
+ )
+ .one()
)
except NoResultFound:
raise KeyError from None
| diff --git a/tests/common/db/packaging.py b/tests/common/db/packaging.py
--- a/tests/common/db/packaging.py
+++ b/tests/common/db/packaging.py
@@ -15,6 +15,7 @@
import factory
import factory.fuzzy
+import packaging.utils
from warehouse.packaging.models import (
BlacklistedProject, Dependency, DependencyKind, File, JournalEntry,
@@ -39,7 +40,9 @@ class Meta:
name = factory.LazyAttribute(lambda o: o.project.name)
project = factory.SubFactory(ProjectFactory)
version = factory.Sequence(lambda n: str(n) + ".0")
- canonical_version = factory.Sequence(lambda n: str(n) + ".0")
+ canonical_version = factory.LazyAttribute(
+ lambda o: packaging.utils.canonicalize_version(o.version)
+ )
_pypi_ordering = factory.Sequence(lambda n: n)
uploader = factory.SubFactory(UserFactory)
diff --git a/tests/unit/packaging/test_models.py b/tests/unit/packaging/test_models.py
--- a/tests/unit/packaging/test_models.py
+++ b/tests/unit/packaging/test_models.py
@@ -73,6 +73,12 @@ def test_traversal_finds(self, db_request):
assert project[release.version] == release
+ def test_traversal_finds_canonical_version(self, db_request):
+ project = DBProjectFactory.create()
+ release = DBReleaseFactory.create(version='1.0', project=project)
+
+ assert project['1.0.0'] == release
+
def test_traversal_cant_find(self, db_request):
project = DBProjectFactory.create()
| Version lookup should take PEP 440 normalization into account
Looking up `1.0.0.0.0` should return `1.0` if it exists, etc.
| We probably don't need this at launch, legacy PyPI doesn't do this (and it'll be easier to do once legacy PyPI is gone since we won't have to implement it twice).
Dustin was just working on version normalization in pypa/packaging#121 so this will be shipped soon. | 2018-03-01T17:53:02Z | [] | [] |
pypi/warehouse | 3,155 | pypi__warehouse-3155 | [
"1000"
] | 9a3b070d381ee0a484d429adcf19665e5ef9b366 | diff --git a/warehouse/email.py b/warehouse/email.py
--- a/warehouse/email.py
+++ b/warehouse/email.py
@@ -19,12 +19,13 @@
@tasks.task(bind=True, ignore_result=True, acks_late=True)
-def send_email(task, request, body, subject, *, recipients=None):
+def send_email(task, request, body, subject, *, recipients=None, bcc=None):
mailer = get_mailer(request)
message = Message(
body=body,
recipients=recipients,
+ bcc=bcc,
sender=request.registry.settings.get('mail.sender'),
subject=subject
)
@@ -145,3 +146,46 @@ def send_primary_email_change_email(request, user, email):
request.task(send_email).delay(body, subject, recipients=[email])
return fields
+
+
+def send_collaborator_added_email(request, user, submitter, project_name, role,
+ email_recipients):
+ fields = {
+ 'username': user.username,
+ 'project': project_name,
+ 'submitter': submitter.username,
+ 'role': role
+ }
+
+ subject = render(
+ 'email/collaborator-added.subject.txt', fields, request=request
+ )
+
+ body = render(
+ 'email/collaborator-added.body.txt', fields, request=request
+ )
+
+ request.task(send_email).delay(body, subject, bcc=email_recipients)
+
+ return fields
+
+
+def send_added_as_collaborator_email(request, submitter, project_name, role,
+ user_email):
+ fields = {
+ 'project': project_name,
+ 'submitter': submitter.username,
+ 'role': role
+ }
+
+ subject = render(
+ 'email/added-as-collaborator.subject.txt', fields, request=request
+ )
+
+ body = render(
+ 'email/added-as-collaborator.body.txt', fields, request=request
+ )
+
+ request.task(send_email).delay(body, subject, recipients=[user_email])
+
+ return fields
diff --git a/warehouse/manage/views.py b/warehouse/manage/views.py
--- a/warehouse/manage/views.py
+++ b/warehouse/manage/views.py
@@ -22,7 +22,8 @@
from warehouse.accounts.models import User, Email
from warehouse.accounts.views import logout
from warehouse.email import (
- send_account_deletion_email, send_email_verification_email,
+ send_account_deletion_email, send_added_as_collaborator_email,
+ send_collaborator_added_email, send_email_verification_email,
send_password_change_email, send_primary_email_change_email
)
from warehouse.manage.forms import (
@@ -551,6 +552,32 @@ def manage_project_roles(project, request, _form_class=CreateRoleForm):
submitted_from=request.remote_addr,
),
)
+
+ owners = (
+ request.db.query(Role)
+ .join(Role.user)
+ .filter(Role.role_name == 'Owner', Role.project == project)
+ )
+ owner_emails = [owner.user.email for owner in owners]
+ owner_emails.remove(request.user.email)
+
+ send_collaborator_added_email(
+ request,
+ user,
+ request.user,
+ project.name,
+ form.role_name.data,
+ owner_emails
+ )
+
+ send_added_as_collaborator_email(
+ request,
+ request.user,
+ project.name,
+ form.role_name.data,
+ user.email
+ )
+
request.session.flash(
f"Added collaborator '{form.username.data}'",
queue="success"
| diff --git a/tests/unit/manage/test_views.py b/tests/unit/manage/test_views.py
--- a/tests/unit/manage/test_views.py
+++ b/tests/unit/manage/test_views.py
@@ -1236,7 +1236,7 @@ def test_post_new_role_validation_fails(self, db_request):
"form": form_obj,
}
- def test_post_new_role(self, db_request):
+ def test_post_new_role(self, monkeypatch, db_request):
project = ProjectFactory.create(name="foobar")
user = UserFactory.create(username="testuser")
@@ -1261,6 +1261,21 @@ def test_post_new_role(self, db_request):
flash=pretend.call_recorder(lambda *a, **kw: None),
)
+ send_collaborator_added_email = pretend.call_recorder(lambda *a: None)
+ monkeypatch.setattr(
+ views,
+ 'send_collaborator_added_email',
+ send_collaborator_added_email,
+ )
+
+ send_added_as_collaborator_email = pretend.call_recorder(
+ lambda *a: None)
+ monkeypatch.setattr(
+ views,
+ 'send_added_as_collaborator_email',
+ send_added_as_collaborator_email,
+ )
+
result = views.manage_project_roles(
project, db_request, _form_class=form_class
)
@@ -1277,6 +1292,26 @@ def test_post_new_role(self, db_request):
pretend.call("Added collaborator 'testuser'", queue="success"),
]
+ assert send_collaborator_added_email.calls == [
+ pretend.call(
+ db_request,
+ user,
+ db_request.user,
+ project.name,
+ form_obj.role_name.data,
+ []
+ )
+ ]
+
+ assert send_added_as_collaborator_email.calls == [
+ pretend.call(
+ db_request,
+ db_request.user,
+ project.name,
+ form_obj.role_name.data,
+ user.email)
+ ]
+
# Only one role is created
role = db_request.db.query(Role).one()
diff --git a/tests/unit/test_email.py b/tests/unit/test_email.py
--- a/tests/unit/test_email.py
+++ b/tests/unit/test_email.py
@@ -384,3 +384,126 @@ def test_primary_email_change_email(
recipients=['old_email'],
),
]
+
+
+class TestCollaboratorAddedEmail:
+
+ def test_collaborator_added_email(
+ self, pyramid_request, pyramid_config, monkeypatch):
+
+ stub_user = pretend.stub(
+ email='email',
+ username='username',
+ )
+ stub_submitter_user = pretend.stub(
+ email='submiteremail',
+ username='submitterusername'
+ )
+ subject_renderer = pyramid_config.testing_add_renderer(
+ 'email/collaborator-added.subject.txt'
+ )
+ subject_renderer.string_response = 'Email Subject'
+ body_renderer = pyramid_config.testing_add_renderer(
+ 'email/collaborator-added.body.txt'
+ )
+ body_renderer.string_response = 'Email Body'
+
+ send_email = pretend.stub(
+ delay=pretend.call_recorder(lambda *args, **kwargs: None)
+ )
+ pyramid_request.task = pretend.call_recorder(
+ lambda *args, **kwargs: send_email
+ )
+ monkeypatch.setattr(email, 'send_email', send_email)
+
+ result = email.send_collaborator_added_email(
+ pyramid_request,
+ user=stub_user,
+ submitter=stub_submitter_user,
+ project_name='test_project',
+ role='Owner',
+ email_recipients=[stub_user.email, stub_submitter_user.email]
+ )
+
+ assert result == {
+ 'username': stub_user.username,
+ 'project': 'test_project',
+ 'role': 'Owner',
+ 'submitter': stub_submitter_user.username
+ }
+ subject_renderer.assert_()
+ body_renderer.assert_(username=stub_user.username)
+ body_renderer.assert_(project='test_project')
+ body_renderer.assert_(role='Owner')
+ body_renderer.assert_(submitter=stub_submitter_user.username)
+
+ assert pyramid_request.task.calls == [
+ pretend.call(send_email),
+ ]
+ assert send_email.delay.calls == [
+ pretend.call(
+ 'Email Body',
+ 'Email Subject',
+ bcc=[stub_user.email, stub_submitter_user.email],
+ ),
+ ]
+
+
+class TestAddedAsCollaboratorEmail:
+
+ def test_added_as_collaborator_email(
+ self, pyramid_request, pyramid_config, monkeypatch):
+
+ stub_user = pretend.stub(
+ email='email',
+ username='username',
+ )
+ stub_submitter_user = pretend.stub(
+ email='submiteremail',
+ username='submitterusername'
+ )
+ subject_renderer = pyramid_config.testing_add_renderer(
+ 'email/added-as-collaborator.subject.txt'
+ )
+ subject_renderer.string_response = 'Email Subject'
+ body_renderer = pyramid_config.testing_add_renderer(
+ 'email/added-as-collaborator.body.txt'
+ )
+ body_renderer.string_response = 'Email Body'
+
+ send_email = pretend.stub(
+ delay=pretend.call_recorder(lambda *args, **kwargs: None)
+ )
+ pyramid_request.task = pretend.call_recorder(
+ lambda *args, **kwargs: send_email
+ )
+ monkeypatch.setattr(email, 'send_email', send_email)
+
+ result = email.send_added_as_collaborator_email(
+ pyramid_request,
+ submitter=stub_submitter_user,
+ project_name='test_project',
+ role='Owner',
+ user_email=stub_user.email
+ )
+
+ assert result == {
+ 'project': 'test_project',
+ 'role': 'Owner',
+ 'submitter': stub_submitter_user.username
+ }
+ subject_renderer.assert_()
+ body_renderer.assert_(submitter=stub_submitter_user.username)
+ body_renderer.assert_(project='test_project')
+ body_renderer.assert_(role='Owner')
+
+ assert pyramid_request.task.calls == [
+ pretend.call(send_email),
+ ]
+ assert send_email.delay.calls == [
+ pretend.call(
+ 'Email Body',
+ 'Email Subject',
+ recipients=[stub_user.email],
+ ),
+ ]
| Email all owners when a new owner/maintainer is added
To make account compromise more obvious, it would be great if all owners of a package were emailed when a new owner or maintainer was added to a package.
In addition, if a user is removed from a role, then that user should also be emailed (eg to prevent an attacker who has compromised one owner, from silently removing other owners of a package, prior to uploading a new malicious package, thereby circumventing #997).
| Thanks for the great suggestion, @edmorley, and sorry for the slow response!
This would be a useful security/audit feature, and in addition, we should also send an email notification to newly added collaborators. And now that we have better email management in Warehouse (just added in the past few weeks), it's far easier to add this feature.
Today in [our development meeting](https://wiki.python.org/psf/PackagingWG/2018-02-20-Warehouse) we discussed where this should go on [our development roadmap](https://wiki.python.org/psf/WarehouseRoadmap). The most urgent task is to improve Warehouse to the point where we can redirect pypi.python.org to pypi.org so the site is more sustainable and reliable. Since this feature isn't something that the legacy site has, I've moved it to a future milestone. But that doesn't have to stop a volunteer from working on it now; if someone wants to write up a pull request for it, go ahead!
Thanks and sorry again for the wait.
I'd be interested to work on this.
I'm not familiar with the codebase yet. Quick look at https://github.com/pypa/warehouse/blob/master/warehouse/manage/views.py#L550, perhaps around there we can send the email to owners. Am I in the right track for this?
Thanks :)
Thanks, @Mariatta! I'll defer to @di who implemented the email management.
@Mariatta Yep, that's the right spot!
I think we should break this up into two emails we send when a new collaborator is added:
* Notification to existing owners that a new collaborator has been added (we probably don't need to send this to the current user).
* Notification to the new collaborator that they have been added. | 2018-03-07T05:23:35Z | [] | [] |
pypi/warehouse | 3,158 | pypi__warehouse-3158 | [
"998"
] | bc48e99c1d4e15b60f52801fcad8b4d1e8edf4fb | diff --git a/warehouse/email.py b/warehouse/email.py
--- a/warehouse/email.py
+++ b/warehouse/email.py
@@ -126,3 +126,23 @@ def send_account_deletion_email(request, user):
request.task(send_email).delay(body, [user.email], subject)
return fields
+
+
+def send_primary_email_change_email(request, user, email):
+ fields = {
+ 'username': user.username,
+ 'old_email': email,
+ 'new_email': user.email,
+ }
+
+ subject = render(
+ 'email/primary-email-change.subject.txt', fields, request=request
+ )
+
+ body = render(
+ 'email/primary-email-change.body.txt', fields, request=request
+ )
+
+ request.task(send_email).delay(body, [email], subject)
+
+ return fields
diff --git a/warehouse/manage/views.py b/warehouse/manage/views.py
--- a/warehouse/manage/views.py
+++ b/warehouse/manage/views.py
@@ -23,7 +23,7 @@
from warehouse.accounts.views import logout
from warehouse.email import (
send_account_deletion_email, send_email_verification_email,
- send_password_change_email,
+ send_password_change_email, send_primary_email_change_email
)
from warehouse.manage.forms import (
AddEmailForm, ChangePasswordForm, CreateRoleForm, ChangeRoleForm,
@@ -163,6 +163,7 @@ def delete_email(self):
request_param=["primary_email_id"],
)
def change_primary_email(self):
+ previous_primary_email = self.request.user.email
try:
new_primary_email = self.request.db.query(Email).filter(
Email.user_id == self.request.user.id,
@@ -187,6 +188,9 @@ def change_primary_email(self):
queue='success',
)
+ send_primary_email_change_email(
+ self.request, self.request.user, previous_primary_email
+ )
return self.default_response
@view_config(
| diff --git a/tests/unit/manage/test_views.py b/tests/unit/manage/test_views.py
--- a/tests/unit/manage/test_views.py
+++ b/tests/unit/manage/test_views.py
@@ -379,8 +379,10 @@ def test_change_primary_email(self, monkeypatch, db_request):
user = UserFactory()
old_primary = EmailFactory(primary=True, user=user)
new_primary = EmailFactory(primary=False, verified=True, user=user)
+ email = EmailFactory(user=user)
db_request.user = user
+
db_request.find_service = lambda *a, **kw: pretend.stub()
db_request.POST = {'primary_email_id': new_primary.id}
db_request.session.flash = pretend.call_recorder(lambda *a, **kw: None)
@@ -389,7 +391,14 @@ def test_change_primary_email(self, monkeypatch, db_request):
)
view = views.ManageAccountViews(db_request)
+ send_email = pretend.call_recorder(lambda *a: None)
+ monkeypatch.setattr(
+ views, 'send_primary_email_change_email', send_email
+ )
assert view.change_primary_email() == view.default_response
+ assert send_email.calls == [
+ pretend.call(db_request, db_request.user, email.email)
+ ]
assert db_request.session.flash.calls == [
pretend.call(
f'Email address {new_primary.email} set as primary.',
diff --git a/tests/unit/test_email.py b/tests/unit/test_email.py
--- a/tests/unit/test_email.py
+++ b/tests/unit/test_email.py
@@ -305,3 +305,50 @@ def test_account_deletion_email(
assert send_email.delay.calls == [
pretend.call('Email Body', [stub_user.email], 'Email Subject'),
]
+
+
+class TestPrimaryEmailChangeEmail:
+
+ def test_primary_email_change_email(
+ self, pyramid_request, pyramid_config, monkeypatch):
+
+ stub_user = pretend.stub(
+ email='new_email',
+ username='username',
+ )
+ subject_renderer = pyramid_config.testing_add_renderer(
+ 'email/primary-email-change.subject.txt'
+ )
+ subject_renderer.string_response = 'Email Subject'
+ body_renderer = pyramid_config.testing_add_renderer(
+ 'email/primary-email-change.body.txt'
+ )
+ body_renderer.string_response = 'Email Body'
+
+ send_email = pretend.stub(
+ delay=pretend.call_recorder(lambda *args, **kwargs: None)
+ )
+ pyramid_request.task = pretend.call_recorder(
+ lambda *args, **kwargs: send_email
+ )
+ monkeypatch.setattr(email, 'send_email', send_email)
+
+ result = email.send_primary_email_change_email(
+ pyramid_request,
+ stub_user,
+ "old_email"
+ )
+
+ assert result == {
+ 'username': stub_user.username,
+ 'old_email': "old_email",
+ 'new_email': stub_user.email
+ }
+ subject_renderer.assert_()
+ body_renderer.assert_(username=stub_user.username)
+ assert pyramid_request.task.calls == [
+ pretend.call(send_email),
+ ]
+ assert send_email.delay.calls == [
+ pretend.call('Email Body', ['old_email'], 'Email Subject'),
+ ]
| Send confirmation when email address changed
Currently on PyPI, if a user changes their contact email address, there is no notification to the old email address, meaning account compromise can go unnoticed.
This means that an attacker can silently prevent other important security notifications (eg #997) from being sent to the real owner.
| Thanks for another great suggestion, @edmorley, and sorry for the slow response!
This would be a very useful security feature, and now that #2898 is written and merged, it's far easier to add this feature.
Today in [our development meeting](https://wiki.python.org/psf/PackagingWG/2018-02-20-Warehouse) we discussed where this should go on [our development roadmap](https://wiki.python.org/psf/WarehouseRoadmap).
Since this feature isn't something that legacy PyPI has, I've moved the issue to a future milestone. But that doesn't have to stop a volunteer from working on it now; if someone wants to write up a pull request for it, go ahead!
Thanks and sorry again for the wait.
I think we should send an email whenever an
- email is added
- email is deleted
- email has been changed to primary email
@brainwane I'm looking into this and will soon put up a PR. | 2018-03-07T14:17:23Z | [] | [] |
pypi/warehouse | 3,165 | pypi__warehouse-3165 | [
"3143"
] | c8ed7650c43ce2df57e99328502a52a7ec6221e1 | diff --git a/warehouse/routes.py b/warehouse/routes.py
--- a/warehouse/routes.py
+++ b/warehouse/routes.py
@@ -178,6 +178,7 @@ def includeme(config):
)
# Packaging
+ config.add_redirect('/p/{name}/', '/project/{name}/', domain=warehouse)
config.add_route(
"packaging.project",
"/project/{name}/",
| diff --git a/tests/unit/test_routes.py b/tests/unit/test_routes.py
--- a/tests/unit/test_routes.py
+++ b/tests/unit/test_routes.py
@@ -269,6 +269,7 @@ def add_policy(name, filename):
]
assert config.add_redirect.calls == [
+ pretend.call("/p/{name}/", "/project/{name}/", domain=warehouse),
pretend.call("/pypi/{name}/", "/project/{name}/", domain=warehouse),
pretend.call(
"/pypi/{name}/{version}/",
| Add a "shortlink" for projects
**From user testing:**
When viewing projects on PyPI, some users type the URL directly if they know the project name.
We should create a shortlink like`pypi.org/p/myproject` which would redirect to `pypi.org/projects/myproject`
cc @di for feedback / guidance.
---
**Good First Issue**: This issue is good for first time contributors. If you've already contributed to Warehouse, please work on [another issue without this label](https://github.com/pypa/warehouse/issues?utf8=%E2%9C%93&q=is%3Aissue+is%3Aopen+-label%3A%22good+first+issue%22) instead. If there is not a corresponding pull request for this issue, it is up for grabs. For directions for getting set up, see our [Getting Started Guide](https://warehouse.pypa.io/development/getting-started/). If you are working on this issue and have questions, please feel free to ask them here, [`#pypa-dev` on Freenode](https://webchat.freenode.net/?channels=%23pypa-dev), or the [pypa-dev mailing list](https://groups.google.com/forum/#!forum/pypa-dev).
| Going to remove the "good first issue" label from this. IMO while this would be nice, it's not likely to happen.
We have a number of other routes that sit at the top level path here, and this would make packages conflict with them, e.g. would https://pypi.org/help/ go to our "Help" page (as it does now) or to the `help` project (currently at https://pypi.org/project/help/)?
We would need to 'audit' the existing routes for conflicting projects and either rename our routes or "reserve" the project name for our own routes.
This would also make adding new routes harder in the future, because we'd need to go through the same process, rather than just adding it as we do now.
What about a short route? E.g. https://pypi.org/p/foobar which would replace, or be an alias to https://pypi.org/project/foobar . It would be a shorter URL to type directly, while not messing up "non project" routes (like https://pypi.org/help).
I'd be fine with a shorter router as an alias. I don't think we gain much by making it the canonical location, but a blanket redirect of ``/p/`` to ``/project/`` seems like it'd be fine, and let people type out something shorter.
Sounds good to me, this is probably a "good first issue" then. I've updated the title/description/labels accordingly. | 2018-03-07T21:45:30Z | [] | [] |
pypi/warehouse | 3,189 | pypi__warehouse-3189 | [
"2861"
] | 744cfbd4fd3f131dc2d78a88307ce47b10658fcc | diff --git a/warehouse/admin/views/users.py b/warehouse/admin/views/users.py
--- a/warehouse/admin/views/users.py
+++ b/warehouse/admin/views/users.py
@@ -23,9 +23,8 @@
from warehouse import forms
from warehouse.accounts.models import User, Email
-from warehouse.packaging.models import JournalEntry, Role
+from warehouse.packaging.models import JournalEntry, Project, Role
from warehouse.utils.paginate import paginate_url_factory
-from warehouse.utils.project import remove_project
@view_config(
@@ -139,16 +138,24 @@ def user_delete(request):
user = request.db.query(User).get(request.matchdict['user_id'])
if user.username != request.params.get('username'):
- print(user.username)
- print(request.params.get('username'))
request.session.flash(f'Wrong confirmation input.', queue='error')
return HTTPSeeOther(
request.route_path('admin.user.detail', user_id=user.id)
)
- # Delete projects one by one so they are purged from the cache
- for project in user.projects:
- remove_project(project, request, flash=False)
+ # Delete all the user's projects
+ projects = (
+ request.db.query(Project)
+ .filter(
+ Project.name.in_(
+ request.db.query(Project.name)
+ .join(Role.project)
+ .filter(Role.user == user)
+ .subquery()
+ )
+ )
+ )
+ projects.delete(synchronize_session=False)
# Update all journals to point to `deleted-user` instead
deleted_user = (
diff --git a/warehouse/cache/origin/__init__.py b/warehouse/cache/origin/__init__.py
--- a/warehouse/cache/origin/__init__.py
+++ b/warehouse/cache/origin/__init__.py
@@ -12,6 +12,8 @@
import collections
import functools
+import operator
+from itertools import chain
from warehouse import db
from warehouse.cache.origin.interfaces import IOriginCache
@@ -87,6 +89,18 @@ def wrapped(context, request):
CacheKeys = collections.namedtuple("CacheKeys", ["cache", "purge"])
+def key_factory(keystring, iterate_on=None):
+
+ def generate_key(obj):
+ if iterate_on:
+ for itr in operator.attrgetter(iterate_on)(obj):
+ yield keystring.format(itr=itr, obj=obj)
+ else:
+ yield keystring.format(obj=obj)
+
+ return generate_key
+
+
def key_maker_factory(cache_keys, purge_keys):
if cache_keys is None:
cache_keys = []
@@ -96,8 +110,14 @@ def key_maker_factory(cache_keys, purge_keys):
def key_maker(obj):
return CacheKeys(
+ # Note: this does not support setting the `cache` argument via
+ # multiple `key_factories` as we do with `purge` because there is
+ # a limit to how many surrogate keys we can attach to a single HTTP
+ # response, and being able to use use `iterate_on` would allow this
+ # size to be unbounded.
+ # ref: https://github.com/pypa/warehouse/pull/3189
cache=[k.format(obj=obj) for k in cache_keys],
- purge=[k.format(obj=obj) for k in purge_keys],
+ purge=chain.from_iterable(key(obj) for key in purge_keys),
)
return key_maker
diff --git a/warehouse/packaging/__init__.py b/warehouse/packaging/__init__.py
--- a/warehouse/packaging/__init__.py
+++ b/warehouse/packaging/__init__.py
@@ -12,6 +12,8 @@
from celery.schedules import crontab
+from warehouse.accounts.models import User
+from warehouse.cache.origin import key_factory
from warehouse.packaging.interfaces import IDownloadStatService, IFileStorage
from warehouse.packaging.services import RedisDownloadStatService
from warehouse.packaging.models import Project, Release
@@ -39,12 +41,28 @@ def includeme(config):
config.register_origin_cache_keys(
Project,
cache_keys=["project/{obj.normalized_name}"],
- purge_keys=["project/{obj.normalized_name}", "all-projects"],
+ purge_keys=[
+ key_factory("project/{obj.normalized_name}"),
+ key_factory("user/{itr.username}", iterate_on='users'),
+ key_factory("all-projects"),
+ ],
)
config.register_origin_cache_keys(
Release,
cache_keys=["project/{obj.project.normalized_name}"],
- purge_keys=["project/{obj.project.normalized_name}", "all-projects"],
+ purge_keys=[
+ key_factory("project/{obj.project.normalized_name}"),
+ key_factory("user/{itr.username}", iterate_on='project.users'),
+ key_factory("all-projects"),
+ ],
+ )
+ config.register_origin_cache_keys(
+ User,
+ cache_keys=["user/{obj.username}"],
+ purge_keys=[
+ key_factory("user/{obj.username}"),
+ key_factory("project/{itr.normalized_name}", iterate_on='projects')
+ ],
)
# Add a periodic task to compute trending once a day, assuming we have
| diff --git a/tests/unit/admin/views/test_users.py b/tests/unit/admin/views/test_users.py
--- a/tests/unit/admin/views/test_users.py
+++ b/tests/unit/admin/views/test_users.py
@@ -18,6 +18,7 @@
from webob.multidict import MultiDict
from warehouse.admin.views import users as views
+from warehouse.packaging.models import Project
from ....common.db.accounts import User, UserFactory, EmailFactory
from ....common.db.packaging import (
@@ -178,6 +179,7 @@ class TestUserDelete:
def test_deletes_user(self, db_request, monkeypatch):
user = UserFactory.create()
project = ProjectFactory.create()
+ another_project = ProjectFactory.create()
journal = JournalEntryFactory(submitted_by=user)
RoleFactory(project=project, user=user, role_name='Owner')
deleted_user = UserFactory.create(username="deleted-user")
@@ -188,17 +190,12 @@ def test_deletes_user(self, db_request, monkeypatch):
db_request.user = UserFactory.create()
db_request.remote_addr = '10.10.10.10'
- remove_project = pretend.call_recorder(lambda *a, **kw: None)
- monkeypatch.setattr(views, 'remove_project', remove_project)
-
result = views.user_delete(db_request)
db_request.db.flush()
assert not db_request.db.query(User).get(user.id)
- assert remove_project.calls == [
- pretend.call(project, db_request, flash=False),
- ]
+ assert db_request.db.query(Project).all() == [another_project]
assert db_request.route_path.calls == [pretend.call('admin.user.list')]
assert result.status_code == 303
assert result.location == '/foobar'
@@ -213,15 +210,12 @@ def test_deletes_user_bad_confirm(self, db_request, monkeypatch):
db_request.params = {'username': 'wrong'}
db_request.route_path = pretend.call_recorder(lambda a, **k: '/foobar')
- remove_project = pretend.call_recorder(lambda *a, **kw: None)
- monkeypatch.setattr(views, 'remove_project', remove_project)
-
result = views.user_delete(db_request)
db_request.db.flush()
assert db_request.db.query(User).get(user.id)
- assert remove_project.calls == []
+ assert db_request.db.query(Project).all() == [project]
assert db_request.route_path.calls == [
pretend.call('admin.user.detail', user_id=user.id),
]
diff --git a/tests/unit/cache/origin/test_init.py b/tests/unit/cache/origin/test_init.py
--- a/tests/unit/cache/origin/test_init.py
+++ b/tests/unit/cache/origin/test_init.py
@@ -204,32 +204,55 @@ class TestKeyMaker:
def test_both_cache_and_purge(self):
key_maker = origin.key_maker_factory(
cache_keys=["foo", "foo/{obj.attr}"],
- purge_keys=["bar", "bar/{obj.attr}"],
- )
- assert key_maker(pretend.stub(attr="bar")) == origin.CacheKeys(
- cache=["foo", "foo/bar"],
- purge=["bar", "bar/bar"],
+ purge_keys=[
+ origin.key_factory("bar"),
+ origin.key_factory("bar/{obj.attr}"),
+ ],
)
+ cache_keys = key_maker(pretend.stub(attr="bar"))
+
+ assert isinstance(cache_keys, origin.CacheKeys)
+ assert cache_keys.cache == ["foo", "foo/bar"]
+ assert list(cache_keys.purge) == ["bar", "bar/bar"]
def test_only_cache(self):
key_maker = origin.key_maker_factory(
cache_keys=["foo", "foo/{obj.attr}"],
purge_keys=None,
)
- assert key_maker(pretend.stub(attr="bar")) == origin.CacheKeys(
- cache=["foo", "foo/bar"],
- purge=[],
- )
+ cache_keys = key_maker(pretend.stub(attr="bar"))
+
+ assert isinstance(cache_keys, origin.CacheKeys)
+ assert cache_keys.cache == ["foo", "foo/bar"]
+ assert list(cache_keys.purge) == []
def test_only_purge(self):
key_maker = origin.key_maker_factory(
cache_keys=None,
- purge_keys=["bar", "bar/{obj.attr}"],
+ purge_keys=[
+ origin.key_factory("bar"),
+ origin.key_factory("bar/{obj.attr}"),
+ ],
)
- assert key_maker(pretend.stub(attr="bar")) == origin.CacheKeys(
- cache=[],
- purge=["bar", "bar/bar"],
+ cache_keys = key_maker(pretend.stub(attr="bar"))
+
+ assert isinstance(cache_keys, origin.CacheKeys)
+ assert cache_keys.cache == []
+ assert list(cache_keys.purge) == ["bar", "bar/bar"]
+
+ def test_iterate_on(self):
+ key_maker = origin.key_maker_factory(
+ cache_keys=['foo'], # Intentionally does not support `iterate_on`
+ purge_keys=[
+ origin.key_factory('bar'),
+ origin.key_factory('bar/{itr}', iterate_on='iterate_me'),
+ ],
)
+ cache_keys = key_maker(pretend.stub(iterate_me=['biz', 'baz']))
+
+ assert isinstance(cache_keys, origin.CacheKeys)
+ assert cache_keys.cache == ['foo']
+ assert list(cache_keys.purge) == ['bar', 'bar/biz', 'bar/baz']
def test_register_origin_keys(monkeypatch):
diff --git a/tests/unit/packaging/test_init.py b/tests/unit/packaging/test_init.py
--- a/tests/unit/packaging/test_init.py
+++ b/tests/unit/packaging/test_init.py
@@ -17,7 +17,7 @@
from warehouse import packaging
from warehouse.packaging.interfaces import IDownloadStatService, IFileStorage
-from warehouse.packaging.models import Project, Release
+from warehouse.packaging.models import Project, Release, User
from warehouse.packaging.tasks import compute_trending
@@ -33,6 +33,11 @@ def test_includme(monkeypatch, with_trending):
packaging, "RedisDownloadStatService", download_stat_service_cls,
)
+ def key_factory(keystring, iterate_on=None):
+ return pretend.call(keystring, iterate_on=iterate_on)
+
+ monkeypatch.setattr(packaging, 'key_factory', key_factory)
+
config = pretend.stub(
maybe_dotted=lambda dotted: storage_class,
register_service=pretend.call_recorder(
@@ -72,14 +77,30 @@ def test_includme(monkeypatch, with_trending):
pretend.call(
Project,
cache_keys=["project/{obj.normalized_name}"],
- purge_keys=["project/{obj.normalized_name}", "all-projects"],
+ purge_keys=[
+ key_factory("project/{obj.normalized_name}"),
+ key_factory("user/{itr.username}", iterate_on='users'),
+ key_factory("all-projects"),
+ ],
),
pretend.call(
Release,
cache_keys=["project/{obj.project.normalized_name}"],
purge_keys=[
- "project/{obj.project.normalized_name}",
- "all-projects",
+ key_factory("project/{obj.project.normalized_name}"),
+ key_factory("user/{itr.username}", iterate_on='project.users'),
+ key_factory("all-projects"),
+ ],
+ ),
+ pretend.call(
+ User,
+ cache_keys=["user/{obj.username}"],
+ purge_keys=[
+ key_factory("user/{obj.username}"),
+ key_factory(
+ "project/{itr.normalized_name}",
+ iterate_on='projects',
+ ),
],
),
]
| Deleting a project should purge user's profile cache
Currently deleting a project does not purge the profile page of the project's owners/maintainers, which contains a link to the project.
| Also, changing the `User` model (e.g., changing the "full name") should also clear the cache for the user's profile. | 2018-03-08T23:36:25Z | [] | [] |
pypi/warehouse | 3,220 | pypi__warehouse-3220 | [
"3089"
] | cb876d0c166edb56c27cd99b93d7592522ca405d | diff --git a/warehouse/accounts/forms.py b/warehouse/accounts/forms.py
--- a/warehouse/accounts/forms.py
+++ b/warehouse/accounts/forms.py
@@ -194,6 +194,15 @@ def __init__(self, *args, user_service, **kwargs):
super().__init__(*args, **kwargs)
self.user_service = user_service
+ def validate_username_or_email(self, field):
+ username_or_email = self.user_service.get_user_by_username(field.data)
+ if username_or_email is None:
+ username_or_email = self.user_service.get_user_by_email(field.data)
+ if username_or_email is None:
+ raise wtforms.validators.ValidationError(
+ "No user found with that username or email"
+ )
+
class ResetPasswordForm(NewPasswordMixin, forms.Form):
diff --git a/warehouse/accounts/views.py b/warehouse/accounts/views.py
--- a/warehouse/accounts/views.py
+++ b/warehouse/accounts/views.py
@@ -282,13 +282,12 @@ def request_password_reset(request, _form_class=RequestPasswordResetForm):
user_service = request.find_service(IUserService, context=None)
form = _form_class(request.POST, user_service=user_service)
-
if request.method == "POST" and form.validate():
user = user_service.get_user_by_username(form.username_or_email.data)
if user is None:
user = user_service.get_user_by_email(form.username_or_email.data)
- if user:
- send_password_reset_email(request, user)
+
+ send_password_reset_email(request, user)
token_service = request.find_service(ITokenService, name='password')
n_hours = token_service.max_age // 60 // 60
diff --git a/warehouse/email.py b/warehouse/email.py
--- a/warehouse/email.py
+++ b/warehouse/email.py
@@ -36,7 +36,6 @@ def send_email(task, request, body, subject, *, recipients=None):
def send_password_reset_email(request, user):
token_service = request.find_service(ITokenService, name='password')
-
token = token_service.dumps({
'action': 'password-reset',
'user.id': str(user.id),
| diff --git a/tests/unit/accounts/test_forms.py b/tests/unit/accounts/test_forms.py
--- a/tests/unit/accounts/test_forms.py
+++ b/tests/unit/accounts/test_forms.py
@@ -365,6 +365,39 @@ def test_no_password_field(self):
form = forms.RequestPasswordResetForm(user_service=user_service)
assert 'password' not in form._fields
+ def test_validate_username_or_email(self):
+ user_service = pretend.stub(
+ get_user_by_username=pretend.call_recorder(lambda userid: "1"),
+ get_user_by_email=pretend.call_recorder(lambda userid: "1"),
+ )
+ form = forms.RequestPasswordResetForm(user_service=user_service)
+ field = pretend.stub(data="username_or_email")
+
+ form.validate_username_or_email(field)
+
+ assert user_service.get_user_by_username.calls == [
+ pretend.call("username_or_email")
+ ]
+
+ def test_validate_username_or_email_with_none(self):
+ user_service = pretend.stub(
+ get_user_by_username=pretend.call_recorder(lambda userid: None),
+ get_user_by_email=pretend.call_recorder(lambda userid: None),
+ )
+ form = forms.RequestPasswordResetForm(user_service=user_service)
+ field = pretend.stub(data="username_or_email")
+
+ with pytest.raises(wtforms.validators.ValidationError):
+ form.validate_username_or_email(field)
+
+ assert user_service.get_user_by_username.calls == [
+ pretend.call("username_or_email")
+ ]
+
+ assert user_service.get_user_by_email.calls == [
+ pretend.call("username_or_email")
+ ]
+
class TestResetPasswordForm:
diff --git a/tests/unit/accounts/test_views.py b/tests/unit/accounts/test_views.py
--- a/tests/unit/accounts/test_views.py
+++ b/tests/unit/accounts/test_views.py
@@ -592,7 +592,9 @@ def test_request_password_reset_with_wrong_credentials(
assert form_class.calls == [
pretend.call(pyramid_request.POST, user_service=user_service),
]
- assert send_password_reset_email.calls == []
+ assert send_password_reset_email.calls == [
+ pretend.call(pyramid_request, None)
+ ]
def test_redirect_authenticated_user(self):
pyramid_request = pretend.stub(authenticated_userid=1)
| Tell user when email is not found when resetting password
Right now if a user tries to recover their account by providing an email address, we don't give any indication whether the email address is actually attached to an account.
Since attempting to register with a already "used" email address will provide the same information (that the email is in use by _some_ PyPI user) there's no real sense in protecting this bit of information here.
Instead we should tell the user that the email was not found, so that they can try a different email they might own, rather than mistakenly thinking that it was the correct email and that the email was not delivered (or that resetting a password is somehow broken).
| @di How about this message? "No user found with that username or email."
@waseem18 Sounds good! | 2018-03-10T20:01:07Z | [] | [] |
pypi/warehouse | 3,236 | pypi__warehouse-3236 | [
"3225"
] | eeabc82178dfcef739df4da09aa37a2aaeefa3e0 | diff --git a/warehouse/packaging/views.py b/warehouse/packaging/views.py
--- a/warehouse/packaging/views.py
+++ b/warehouse/packaging/views.py
@@ -122,16 +122,21 @@ def release_detail(release, request):
)
]
- # Get the license from the classifiers or metadata, preferring classifiers.
- license = None
- if release.license:
- # Make a best effort when the entire license text is given
- # by using the first line only.
- license = release.license.split('\n')[0]
- license_classifiers = [c.split(" :: ")[-1] for c in release.classifiers
- if c.startswith("License")]
- if license_classifiers:
- license = ', '.join(license_classifiers)
+ # Get the license from both the `Classifier` and `License` metadata fields
+ license_classifiers = ', '.join(
+ c.split(" :: ")[-1]
+ for c in release.classifiers
+ if c.startswith("License")
+ )
+
+ # Make a best effort when the entire license text is given by using the
+ # first line only.
+ short_license = release.license.split('\n')[0] if release.license else None
+
+ if license_classifiers and short_license:
+ license = f'{license_classifiers} ({short_license})'
+ else:
+ license = license_classifiers or short_license or None
return {
"project": project,
| diff --git a/tests/unit/packaging/test_views.py b/tests/unit/packaging/test_views.py
--- a/tests/unit/packaging/test_views.py
+++ b/tests/unit/packaging/test_views.py
@@ -206,11 +206,11 @@ def test_license_from_classifier(self, db_request):
classifier="License :: OSI Approved :: BSD License")
release = ReleaseFactory.create(
_classifiers=[other_classifier, classifier],
- license="Will not be used")
+ license="Will be added at the end")
result = views.release_detail(release, db_request)
- assert result["license"] == "BSD License"
+ assert result["license"] == "BSD License (Will be added at the end)"
def test_license_with_no_classifier(self, db_request):
"""With no classifier, a license is used from metadata."""
| License metadata seems to be ignored
When I made the latest release of `python-dateutil`, it came along with a license change from BSD to Apache / BSD dual licensed. I updated the `license=` metadata in `setup.py`, but I forgot to update the trove classifiers.
[The page on PyPI](https://pypi.python.org/pypi/python-dateutil/2.7.0) shows the license as "Apache 2.0" as I would expect. [The page on warehouse](https://pypi.org/project/python-dateutil/) shows the license as "BSD License". I'm assuming it's pulling that from the trove classifier? Shouldn't it pull it from the `license` field if that is populated?
| 2018-03-12T23:40:44Z | [] | [] |
|
pypi/warehouse | 3,253 | pypi__warehouse-3253 | [
"2649"
] | fbc5c79331d025890f073faac27f2c530596de2e | diff --git a/warehouse/admin/routes.py b/warehouse/admin/routes.py
--- a/warehouse/admin/routes.py
+++ b/warehouse/admin/routes.py
@@ -91,6 +91,18 @@ def includeme(config):
domain=warehouse,
)
+ # Classifier related Admin pages
+ config.add_route(
+ 'admin.classifiers',
+ '/admin/classifiers/',
+ domain=warehouse,
+ )
+ config.add_route(
+ 'admin.classifiers.add',
+ '/admin/classifiers/add/',
+ domain=warehouse,
+ )
+
# Blacklist related Admin pages
config.add_route(
"admin.blacklist.list",
diff --git a/warehouse/admin/views/classifiers.py b/warehouse/admin/views/classifiers.py
new file mode 100644
--- /dev/null
+++ b/warehouse/admin/views/classifiers.py
@@ -0,0 +1,69 @@
+# Licensed under the Apache License, Version 2.0 (the "License");
+# you may not use this file except in compliance with the License.
+# You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing, software
+# distributed under the License is distributed on an "AS IS" BASIS,
+# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+# See the License for the specific language governing permissions and
+# limitations under the License.
+
+from pyramid.httpexceptions import HTTPSeeOther
+from pyramid.view import view_config
+
+from warehouse.packaging.models import Classifier
+
+
+@view_config(
+ route_name="admin.classifiers",
+ renderer="admin/classifiers/index.html",
+ permission="admin",
+ uses_session=True,
+)
+def get_classifiers(request):
+ classifiers = (
+ request.db.query(Classifier)
+ .filter(Classifier.l5 == 0) # Can be a parent
+ .order_by(Classifier.classifier)
+ .all()
+ )
+
+ return {
+ 'classifiers': classifiers,
+ }
+
+
+@view_config(
+ route_name="admin.classifiers.add",
+ permission="admin",
+ request_method="POST",
+ uses_session=True,
+ require_methods=False,
+ require_csrf=True,
+)
+def add_classifier(request):
+ parent = request.db.query(Classifier).get(request.params.get('parent_id'))
+
+ classifier = Classifier(
+ l2=parent.l2,
+ l3=parent.l3,
+ l4=parent.l4,
+ l5=parent.l5,
+ classifier=parent.classifier + " :: " + request.params.get('child'),
+ )
+ request.db.add(classifier)
+ request.db.flush() # To get the ID
+
+ for level in ['l3', 'l4', 'l5']:
+ if getattr(classifier, level) == 0:
+ setattr(classifier, level, classifier.id)
+ break
+
+ request.session.flash(
+ f"Successfully added classifier {classifier.classifier!r}",
+ queue="success",
+ )
+
+ return HTTPSeeOther(request.route_path("admin.classifiers"))
diff --git a/warehouse/migrations/versions/2730e54f8717_reset_classifier_id_sequence.py b/warehouse/migrations/versions/2730e54f8717_reset_classifier_id_sequence.py
new file mode 100644
--- /dev/null
+++ b/warehouse/migrations/versions/2730e54f8717_reset_classifier_id_sequence.py
@@ -0,0 +1,35 @@
+# Licensed under the Apache License, Version 2.0 (the "License");
+# you may not use this file except in compliance with the License.
+# You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing, software
+# distributed under the License is distributed on an "AS IS" BASIS,
+# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+# See the License for the specific language governing permissions and
+# limitations under the License.
+"""
+Reset Classifier ID sequence
+
+Revision ID: 2730e54f8717
+Revises: 8fd3400c760f
+Create Date: 2018-03-14 16:34:38.151300
+"""
+
+from alembic import op
+
+
+revision = '2730e54f8717'
+down_revision = '8fd3400c760f'
+
+
+def upgrade():
+ op.execute("""
+ SELECT setval('trove_classifiers_id_seq', max(id))
+ FROM trove_classifiers;
+ """)
+
+
+def downgrade():
+ pass
| diff --git a/tests/unit/admin/test_routes.py b/tests/unit/admin/test_routes.py
--- a/tests/unit/admin/test_routes.py
+++ b/tests/unit/admin/test_routes.py
@@ -91,6 +91,16 @@ def test_includeme():
"/admin/journals/",
domain=warehouse,
),
+ pretend.call(
+ 'admin.classifiers',
+ '/admin/classifiers/',
+ domain=warehouse,
+ ),
+ pretend.call(
+ 'admin.classifiers.add',
+ '/admin/classifiers/add/',
+ domain=warehouse,
+ ),
pretend.call(
"admin.blacklist.list",
"/admin/blacklist/",
diff --git a/tests/unit/admin/views/test_classifiers.py b/tests/unit/admin/views/test_classifiers.py
new file mode 100644
--- /dev/null
+++ b/tests/unit/admin/views/test_classifiers.py
@@ -0,0 +1,72 @@
+# Licensed under the Apache License, Version 2.0 (the "License");
+# you may not use this file except in compliance with the License.
+# You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing, software
+# distributed under the License is distributed on an "AS IS" BASIS,
+# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+# See the License for the specific language governing permissions and
+# limitations under the License.
+
+import pretend
+import pytest
+
+from warehouse.admin.views import classifiers as views
+from warehouse.classifiers.models import Classifier
+
+from ....common.db.classifiers import ClassifierFactory
+
+
+class TestGetClassifiers:
+
+ def test_get_classifiers(self, db_request):
+ classifier_a = ClassifierFactory(l5=0, classifier='I am first')
+ classifier_b = ClassifierFactory(l5=0, classifier='I am last')
+ ClassifierFactory(l5=1) # Ignored because it has a nonzero L5
+
+ assert views.get_classifiers(db_request) == {
+ 'classifiers': [classifier_a, classifier_b],
+ }
+
+
+class TestAddClassifier:
+
+ @pytest.mark.parametrize(
+ 'parent_levels, expected_levels',
+ [
+ ((2, 0, 0, 0), (2, None, 0, 0)),
+ ((2, 3, 0, 0), (2, 3, None, 0)),
+ ((2, 3, 4, 0), (2, 3, 4, None)),
+
+ # This won't actually happen but it's needed for coverage
+ ((2, 3, 4, 5), (2, 3, 4, 5)),
+ ]
+ )
+ def test_add_classifier(self, db_request, parent_levels, expected_levels):
+ l2, l3, l4, l5 = parent_levels
+ parent = ClassifierFactory(
+ l2=l2, l3=l3, l4=l4, l5=l5, classifier='Parent'
+ )
+
+ db_request.params = {
+ 'parent_id': parent.id,
+ 'child': 'Foobar',
+ }
+ db_request.session.flash = pretend.call_recorder(lambda *a, **kw: None)
+ db_request.route_path = lambda *a: '/the/path'
+
+ views.add_classifier(db_request)
+
+ new = (
+ db_request.db.query(Classifier)
+ .filter(Classifier.classifier == 'Parent :: Foobar')
+ .one()
+ )
+
+ new_l2, new_l3, new_l4, new_l5 = expected_levels
+ assert new.l2 == new_l2 if new_l2 is not None else new.id
+ assert new.l3 == new_l3 if new_l3 is not None else new.id
+ assert new.l4 == new_l4 if new_l4 is not None else new.id
+ assert new.l5 == new_l5 if new_l5 is not None else new.id
| Ability to add new classifiers.
On the admin side of things, we need a simple way to add new classifiers.
| In our [meeting today](https://wiki.python.org/psf/PackagingWG/2018-02-12-Warehouse) Ernest said that this is something he would like in the admin UI before launch.
@lgh2 is about to start working on this.
In IRC conversation we found out Dustin had already started working on this, so now it's on his plate. :) | 2018-03-14T17:45:12Z | [] | [] |
pypi/warehouse | 3,273 | pypi__warehouse-3273 | [
"1299"
] | 4a9cd7735b60c7803cd9d6c6caa77de03fccdc5c | diff --git a/warehouse/views.py b/warehouse/views.py
--- a/warehouse/views.py
+++ b/warehouse/views.py
@@ -318,6 +318,36 @@ def filter_key(item):
except ValueError:
return 1, 0, item[0]
+ def form_filters_tree(split_list):
+ """
+ Takes a list of lists, each of them containing a filter and
+ one of its children.
+ Returns a dictionary, each key being a filter and each value being
+ the filter's children.
+ """
+ d = {}
+ for l in split_list:
+ current_level = d
+ for part in l:
+ if part not in current_level:
+ current_level[part] = {}
+ current_level = current_level[part]
+ return d
+
+ def process_available_filters():
+ """
+ Processes available filters and returns a list of dictionaries.
+ The value of a key in the dictionary represents its children
+ """
+ sorted_filters = sorted(available_filters.items(), key=filter_key)
+ output = []
+ for f in sorted_filters:
+ classifier_list = f[1]
+ split_list = [i.split(" :: ") for i in classifier_list]
+ tree = form_filters_tree(split_list)
+ output.append(tree)
+ return output
+
metrics = request.find_service(IMetricsService, context=None)
metrics.histogram("warehouse.views.search.results", page.item_count)
@@ -325,7 +355,7 @@ def filter_key(item):
"page": page,
"term": q,
"order": request.params.get("o", ""),
- "available_filters": sorted(available_filters.items(), key=filter_key),
+ "available_filters": process_available_filters(),
"applied_filters": request.params.getall("c"),
}
| diff --git a/tests/unit/test_views.py b/tests/unit/test_views.py
--- a/tests/unit/test_views.py
+++ b/tests/unit/test_views.py
@@ -441,7 +441,12 @@ def test_with_classifiers(self, monkeypatch, db_request, metrics, page):
"order": params.get("o", ""),
"applied_filters": params.getall("c"),
"available_filters": [
- ("foo", [classifier1.classifier, classifier2.classifier])
+ {
+ "foo": {
+ classifier1.classifier.split(" :: ")[1]: {},
+ classifier2.classifier.split(" :: ")[1]: {},
+ }
+ }
],
}
assert ("fiz", [classifier3.classifier]) not in search_view["available_filters"]
| Build tree for trove classifiers
Ref #1265 and #754
We need to build a new SCSS block that renders as a tree, as per http://odyniec.net/articles/turning-lists-into-trees/
This should be applied to the trove classifiers in the search sidebar and on the package detail pages.
This will require the markup for each to change to a unordered list.
| Visual Spec (with checkboxes):

The standard tree without checkboxes, would be the same, but with the lines joining instead of making boxes.
@nlhkabu I'm looking into this issue - Right now going through http://odyniec.net/articles/turning-lists-into-trees/. Will put a WIP PR after I make some progress on this. | 2018-03-15T20:37:58Z | [] | [] |
pypi/warehouse | 3,292 | pypi__warehouse-3292 | [
"3284"
] | 7dcff19af4882c4e18bb7456fb2504cfdf08b13a | diff --git a/warehouse/legacy/api/simple.py b/warehouse/legacy/api/simple.py
--- a/warehouse/legacy/api/simple.py
+++ b/warehouse/legacy/api/simple.py
@@ -87,7 +87,7 @@ def simple_detail(project, request):
)
)
.all(),
- key=lambda f: (parse(f.version), f.packagetype)
+ key=lambda f: (parse(f.version), f.filename)
)
return {"project": project, "files": files}
| diff --git a/tests/unit/legacy/api/test_simple.py b/tests/unit/legacy/api/test_simple.py
--- a/tests/unit/legacy/api/test_simple.py
+++ b/tests/unit/legacy/api/test_simple.py
@@ -202,7 +202,7 @@ def test_with_files_with_version_multi_digit(self, db_request):
files = []
for files_release in \
- zip(egg_files, wheel_files, tar_files):
+ zip(egg_files, tar_files, wheel_files):
files += files_release
db_request.matchdict["name"] = project.normalized_name
@@ -212,9 +212,6 @@ def test_with_files_with_version_multi_digit(self, db_request):
# Make sure that we get any changes made since the JournalEntry was
# saved.
db_request.db.refresh(project)
- import pprint
- pprint.pprint(simple.simple_detail(project, db_request)['files'])
- pprint.pprint(files)
assert simple.simple_detail(project, db_request) == {
"project": project,
| Warehouse file order differs from legacy PyPI file list
Tonight, while load testing of pypi.org was ongoing, we saw some failures in automated systems that use `--require-hashes` with `pip install`, as ordering on the package file list page changed.
The specific package we saw break was `pandas` at version `0.12.0`. We had a single hash for `pandas-0.12.0.tar.gz`. A few of our hosts were served from the legacy PyPI service, which succeeded as normal. The Warehouse endpoint, however, failed, since `pandas-0.12.0.zip` now preceded `pandas-0.12.0.tar.gz` in the file list.
At the moment, you can see that https://pypi.org/simple/pandas/ and https://pypi.python.org/simple/pandas/ differ by searching for `pandas-0.12.0.tar.gz` and `pandas-0.12.0.zip` and comparing the position.
| Could you tell us what version of `pip` was used, and if you were using an intermediary or mirrored index? The order of the distributions shouldn't cause an issue.
We were using `pip` version 9.0.1 with no explicit index specified, so the default `https://pypi.python.org/simple`.
I can reproduce this currently with a local Python 2.7 install:
```
$ pip --version
pip 9.0.1
```
```
$ pip install --index-url https://pypi.python.org/simple pandas==0.12.0
Collecting pandas==0.12.0
Downloading pandas-0.12.0.tar.gz (3.2MB)
```
```
$ pip install --index-url https://pypi.org/simple pandas==0.12.0
Collecting pandas==0.12.0
Downloading https://files.pythonhosted.org/packages/49/32/61120c7350105faa1533211fe3226ca6e71cd08a50e6e137e0baee50d186/pandas-0.12.0.zip (3.4MB)
```
From a cursory look at [_link_package_versions()](https://github.com/pypa/pip/blob/9.0.1/pip/index.py#L605) it seems that the ordering between `.zip` and `.tar.gz` ultimately does come down to the order on the links page.
Yea, pip respects the order files are given to it by the index as the final tie breaker when it sorts files, so the order in ``/simple/`` can change what file gets installed. | 2018-03-16T14:37:12Z | [] | [] |
pypi/warehouse | 3,310 | pypi__warehouse-3310 | [
"2490",
"2928"
] | 99d377b1ba2df69e6edfd3347db33a55d8b9b6e1 | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -660,7 +660,9 @@ def _is_valid_dist_file(filename, filetype):
def _is_duplicate_file(db_session, filename, hashes):
"""
- Check to see if file already exists, and if it's content matches
+ Check to see if file already exists, and if it's content matches.
+ A file is considered to exist if its filename *or* blake2 digest are
+ present in a file row in the database.
Returns:
- True: This file is a duplicate and all further processing should halt.
@@ -670,14 +672,19 @@ def _is_duplicate_file(db_session, filename, hashes):
file_ = (
db_session.query(File)
- .filter(File.filename == filename)
+ .filter(
+ (File.filename == filename) |
+ (File.blake2_256_digest == hashes["blake2_256"]))
.first()
)
if file_ is not None:
- return (file_.sha256_digest == hashes["sha256"] and
- file_.md5_digest == hashes["md5"] and
- file_.blake2_256_digest == hashes["blake2_256"])
+ return (
+ file_.filename == filename and
+ file_.sha256_digest == hashes["sha256"] and
+ file_.md5_digest == hashes["md5"] and
+ file_.blake2_256_digest == hashes["blake2_256"]
+ )
return None
@@ -1075,7 +1082,7 @@ def file_upload(request):
return Response()
elif is_duplicate is not None:
raise _exc_with_message(
- HTTPBadRequest, "File already exists. "
+ HTTPBadRequest, "The filename or contents already exist. "
"See " +
request.route_url(
'help', _anchor='file-name-reuse'
| diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -639,10 +639,52 @@ def test_is_duplicate_none(self, pyramid_config, db_request):
),
)
+ hashes["blake2_256"] = "another blake2 digest"
+
assert legacy._is_duplicate_file(
db_request.db, requested_file_name, hashes
) is None
+ def test_is_duplicate_false_same_blake2(self, pyramid_config, db_request):
+ pyramid_config.testing_securitypolicy(userid=1)
+
+ user = UserFactory.create()
+ EmailFactory.create(user=user)
+ project = ProjectFactory.create()
+ release = ReleaseFactory.create(project=project, version="1.0")
+ RoleFactory.create(user=user, project=project)
+
+ filename = "{}-{}.tar.gz".format(project.name, release.version)
+ requested_file_name = "{}-{}-1.tar.gz".format(project.name,
+ release.version)
+ file_content = io.BytesIO(b"A fake file.")
+ file_value = file_content.getvalue()
+
+ hashes = {
+ "sha256": hashlib.sha256(file_value).hexdigest(),
+ "md5": hashlib.md5(file_value).hexdigest(),
+ "blake2_256": hashlib.blake2b(
+ file_value, digest_size=256 // 8
+ ).hexdigest()
+ }
+ db_request.db.add(
+ File(
+ release=release,
+ filename=filename,
+ md5_digest=hashes["md5"],
+ sha256_digest=hashes["sha256"],
+ blake2_256_digest=hashes["blake2_256"],
+ path="source/{name[0]}/{name}/{filename}".format(
+ name=project.name,
+ filename=filename,
+ ),
+ ),
+ )
+
+ assert legacy._is_duplicate_file(
+ db_request.db, requested_file_name, hashes
+ ) is False
+
def test_is_duplicate_false(self, pyramid_config, db_request):
pyramid_config.testing_securitypolicy(userid=1)
@@ -1792,7 +1834,69 @@ def test_upload_fails_with_existing_filename_diff_content(self,
]
assert resp.status_code == 400
assert resp.status == (
- "400 File already exists. "
+ "400 The filename or contents already exist. "
+ "See /the/help/url/"
+ )
+
+ def test_upload_fails_with_diff_filename_same_blake2(self,
+ pyramid_config,
+ db_request):
+ pyramid_config.testing_securitypolicy(userid=1)
+
+ user = UserFactory.create()
+ project = ProjectFactory.create()
+ release = ReleaseFactory.create(project=project, version="1.0")
+ RoleFactory.create(user=user, project=project)
+
+ filename = "{}-{}.tar.gz".format(project.name, release.version)
+ file_content = io.BytesIO(b"A fake file.")
+
+ db_request.POST = MultiDict({
+ "metadata_version": "1.2",
+ "name": project.name,
+ "version": release.version,
+ "filetype": "sdist",
+ "md5_digest": hashlib.md5(file_content.getvalue()).hexdigest(),
+ "content": pretend.stub(
+ filename="{}-fake.tar.gz".format(project.name),
+ file=file_content,
+ type="application/tar",
+ ),
+ })
+
+ db_request.db.add(
+ File(
+ release=release,
+ filename=filename,
+ md5_digest=hashlib.md5(file_content.getvalue()).hexdigest(),
+ sha256_digest=hashlib.sha256(
+ file_content.getvalue()
+ ).hexdigest(),
+ blake2_256_digest=hashlib.blake2b(
+ file_content.getvalue(),
+ digest_size=256 // 8
+ ).hexdigest(),
+ path="source/{name[0]}/{name}/{filename}".format(
+ name=project.name,
+ filename=filename,
+ ),
+ ),
+ )
+ db_request.route_url = pretend.call_recorder(
+ lambda route, **kw: "/the/help/url/"
+ )
+
+ with pytest.raises(HTTPBadRequest) as excinfo:
+ legacy.file_upload(db_request)
+
+ resp = excinfo.value
+
+ assert db_request.route_url.calls == [
+ pretend.call('help', _anchor='file-name-reuse')
+ ]
+ assert resp.status_code == 400
+ assert resp.status == (
+ "400 The filename or contents already exist. "
"See /the/help/url/"
)
| Validate Blake2 Uniqueness prior to saving
For some reason sometimes people are uploading files that already exist with different filenames. I'm not sure if there is a valid use case for this, but if there is we need to either remove the ``UNIQUE`` constraint on the blake2 hash or if there isn't we should validate this before we attempt to save the row.
---
https://sentry.io/python-software-foundation/warehouse-production/issues/147880375/
```
IntegrityError: duplicate key value violates unique constraint "release_files_blake2_256_digest_key"
DETAIL: Key (blake2_256_digest)=(1d31e5fd783d7ce96471b6d66ba3dd59e3e0722811ecbf5c19173a5194be514c) already exists.
File "sqlalchemy/engine/base.py", line 1182, in _execute_context
context)
File "sqlalchemy/engine/default.py", line 470, in do_execute
cursor.execute(statement, parameters)
IntegrityError: (psycopg2.IntegrityError) duplicate key value violates unique constraint "release_files_blake2_256_digest_key"
DETAIL: Key (blake2_256_digest)=(1d31e5fd783d7ce96471b6d66ba3dd59e3e0722811ecbf5c19173a5194be514c) already exists.
[SQL: 'INSERT INTO release_files (name, version, python_version, requires_python, packagetype, comment_text, filename, path, size, has_signature, md5_digest, sha256_digest, blake2_256_digest) VALUES (%(name)s, %(version)s, %(python_version)s, %(requires_python)s, %(packagetype)s, %(comment_text)s, %(filename)s, %(path)s, %(size)s, %(has_signature)s, %(md5_digest)s, %(sha256_digest)s, %(blake2_256_digest)s) RETURNING release_files.id'] [parameters: {'name': 'treelite', 'version': '0.1a1', 'python_version': 'cp27', 'requires_python': None, 'packagetype': 'bdist_wheel', 'comment_text': '', 'filename': 'treelite-0.1a3-cp27-cp27m-macosx_10_6_intel.whl', 'path': '1d/31/e5fd783d7ce96471b6d66ba3dd59e3e0722811ecbf5c19173a5194be514c/treelite-0.1a3-cp27-cp27m-macosx_10_6_intel.whl', 'size': 1574670, 'has_signature': False, 'md5_digest': '32229792ce7968ecd29f9b099cb69644', 'sha256_digest': 'eea762a38b556c0a1f76640be5fcea73eb897d67dc5006dbe590162fbdc5bdea', 'blake2_256_digest': '1d31e5fd783d7ce96471b6d66ba3dd59e3e0722811ecbf5c19173a5194be514c'}]
(44 additional frame(s) were not displayed)
...
File "warehouse/config.py", line 85, in require_https_tween
return handler(request)
File "warehouse/static.py", line 78, in whitenoise_tween
return handler(request)
File "warehouse/utils/compression.py", line 93, in compression_tween
response = handler(request)
File "warehouse/raven.py", line 41, in raven_tween
return handler(request)
File "warehouse/cache/http.py", line 69, in conditional_http_tween
response = handler(request)
IntegrityError: (psycopg2.IntegrityError) duplicate key value violates unique constraint "release_files_blake2_256_digest_key"
DETAIL: Key (blake2_256_digest)=(1d31e5fd783d7ce96471b6d66ba3dd59e3e0722811ecbf5c19173a5194be514c) already exists.
[SQL: 'INSERT INTO release_files (name, version, python_version, requires_python, packagetype, comment_text, filename, path, size, has_signature, md5_digest, sha256_digest, blake2_256_digest) VALUES (%(name)s, %(version)s, %(python_version)s, %(requires_python)s, %(packagetype)s, %(comment_text)s, %(filename)s, %(path)s, %(size)s, %(has_signature)s, %(md5_digest)s, %(sha256_digest)s, %(blake2_256_digest)s) RETURNING release_files.id'] [parameters: {'name': 'treelite', 'version': '0.1a1', 'python_version': 'cp27', 'requires_python': None, 'packagetype': 'bdist_wheel', 'comment_text': '', 'filename': 'treelite-0.1a3-cp27-cp27m-macosx_10_6_intel.whl', 'path': '1d/31/e5fd783d7ce96471b6d66ba3dd59e3e0722811ecbf5c19173a5194be514c/treelite-0.1a3-cp27-cp27m-macosx_10_6_intel.whl', 'size': 1574670, 'has_signature': False, 'md5_digest': '32229792ce7968ecd29f9b099cb69644', 'sha256_digest': 'eea762a38b556c0a1f76640be5fcea73eb897d67dc5006dbe590162fbdc5bdea', 'blake2_256_digest': '1d31e5fd783d7ce96471b6d66ba3dd59e3e0722811ecbf5c19173a5194be514c'}]
```
Check if another file has been uploaded with the same blake2 digest
This tries to resolve #2490
I'm not sure if the uniqueness of the blake2 digest should be validated on file uploading or before, or if hijacking on the same "File already exists" message is helpful enough.
Also, I'm getting a weird error on my test, making it fail. Something about the request object missing a 'user' attribute.
Thanks for your feedback y'all.
🐍
|
The error is because your test is getting to this line:
```python
request.db.add(
JournalEntry(
name=release.project.name,
version=release.version,
action="add {python_version} file {filename}".format(
python_version=file_.python_version,
filename=file_.filename,
),
> submitted_by=request.user,
submitted_from=request.remote_addr,
),
)
```
Which means it's trying to add the file being uploaded, so it seems like either something is wrong with your test, or with the new logic you added.
Hey @alanbato, need any help or more direction here?
I think @alanbato [is having computer problems](https://twitter.com/alanbato/status/972266164855410688).
Yes I am 😢
If anyone wants to continue and build on this PR, please do so. I'll ping this thread when my computer gets better 😓 | 2018-03-18T19:00:15Z | [] | [] |
pypi/warehouse | 3,314 | pypi__warehouse-3314 | [
"3186"
] | 57e383fc97f679a3308a2ff4e5e994d264c03e8a | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -813,10 +813,12 @@ def file_upload(request):
func.normalize_pep426_name(form.name.data))).scalar():
raise _exc_with_message(
HTTPBadRequest,
- ("The name {!r} is not allowed. "
- "See https://pypi.org/help/#project-name "
- "for more information.")
- .format(form.name.data),
+ ("The name {name!r} is not allowed. "
+ "See {projecthelp} "
+ "for more information.").format(
+ name=form.name.data,
+ projecthelp=request.route_url(
+ 'help', _anchor='project-name')),
) from None
# Also check for collisions with Python Standard Library modules.
| diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -915,14 +915,22 @@ def test_fails_with_invalid_names(self, pyramid_config, db_request, name):
),
})
+ db_request.route_url = pretend.call_recorder(
+ lambda route, **kw: "/the/help/url/"
+ )
+
with pytest.raises(HTTPBadRequest) as excinfo:
legacy.file_upload(db_request)
resp = excinfo.value
+ assert db_request.route_url.calls == [
+ pretend.call('help', _anchor='project-name')
+ ]
+
assert resp.status_code == 400
assert resp.status == ("400 The name {!r} is not allowed. "
- "See https://pypi.org/help/#project-name "
+ "See /the/help/url/ "
"for more information.").format(name)
@pytest.mark.parametrize("name", ["xml", "XML", "pickle", "PiCKle",
| Make error message (for invalid project name) work with Test PyPI
Right now, when someone tries to upload a package to PyPI but the project name they want to use is not a project name we allow, the error message we send them includes -- hardcoded -- the URL https://pypi.org. Instead, we should use Pyramid's URL routing to refer to the URL of the PyPI in question, thus ensuring that we send error messages that, as appropriate, refer to pypi.org, test.pypi.org, or any other future PyPI.
To fix this, look at `warehouse/forklift/legacy.py` and `tests/unit/forklift/test_legacy.py` ([the test is `test_fails_with_invalid_names`](https://github.com/pypa/warehouse/blob/master/tests/unit/forklift/test_legacy.py#L872)), and make changes similar to the changes I made in #3183. If you're used to string substitution/string formatting in Python, this should be a relatively approachable first Warehouse issue.
---
**Good First Issue**: This issue is good for first time contributors. If you've already contributed to Warehouse, please work on [another issue without this label](https://github.com/pypa/warehouse/issues?utf8=%E2%9C%93&q=is%3Aissue+is%3Aopen+-label%3A%22good+first+issue%22) instead. If there is not a corresponding pull request for this issue, it is up for grabs. For directions for getting set up, see our [Getting Started Guide](https://warehouse.pypa.io/development/getting-started/). If you are working on this issue and have questions, please feel free to ask them here, [`#pypa-dev` on Freenode](https://webchat.freenode.net/?channels=%23pypa-dev), or the [pypa-dev mailing list](https://groups.google.com/forum/#!forum/pypa-dev).
| I'm working on this issue.
Hi @pgadige! Saw your question in IRC about logging in within your local developer environment. As Dustin said, check https://warehouse.readthedocs.io/development/getting-started/#logging-in-to-warehouse . Glad to have you joining us!
You mentioned you're interested in the larger process of building something like Warehouse. I recommend you take a look at:
* [the PyPA's list of presentations and articles](https://www.pypa.io/en/latest/presentations/)
* [PyPA's history of Python packaging](https://www.pypa.io/en/latest/history/)
* [the Warehouse roadmap](https://wiki.python.org/psf/WarehouseRoadmap)
* [blog posts, mailing list messages, and notes from our core developer meetings](https://wiki.python.org/psf/PackagingWG)
* [Warehouse application overview](https://warehouse.readthedocs.io/application/) | 2018-03-19T19:23:09Z | [] | [] |
pypi/warehouse | 3,339 | pypi__warehouse-3339 | [
"3174"
] | 8d744af2dc6d1b510063ce647a39b58635c905cd | diff --git a/warehouse/accounts/forms.py b/warehouse/accounts/forms.py
--- a/warehouse/accounts/forms.py
+++ b/warehouse/accounts/forms.py
@@ -14,7 +14,7 @@
import wtforms
import wtforms.fields.html5
-from warehouse import forms, recaptcha
+from warehouse import forms
from warehouse.accounts.interfaces import TooManyFailedLogins
@@ -155,27 +155,22 @@ def validate_email(self, field):
)
+class HoneypotMixin:
+
+ """ A mixin to catch spammers. This field should always be blank """
+
+ confirm_form = wtforms.StringField()
+
+
class RegistrationForm(
- NewUsernameMixin, NewEmailMixin, NewPasswordMixin, forms.Form):
+ NewUsernameMixin, NewEmailMixin, NewPasswordMixin, HoneypotMixin,
+ forms.Form):
full_name = wtforms.StringField()
- g_recaptcha_response = wtforms.StringField()
- def __init__(self, *args, recaptcha_service, user_service, **kwargs):
+ def __init__(self, *args, user_service, **kwargs):
super().__init__(*args, **kwargs)
self.user_service = user_service
- self.recaptcha_service = recaptcha_service
-
- def validate_g_recaptcha_response(self, field):
- # do required data validation here due to enabled flag being required
- if self.recaptcha_service.enabled and not field.data:
- raise wtforms.validators.ValidationError("Recaptcha error.")
- try:
- self.recaptcha_service.verify_response(field.data)
- except recaptcha.RecaptchaError:
- # TODO: log error
- # don't want to provide the user with any detail
- raise wtforms.validators.ValidationError("Recaptcha error.")
class LoginForm(PasswordMixin, UsernameMixin, forms.Form):
diff --git a/warehouse/accounts/views.py b/warehouse/accounts/views.py
--- a/warehouse/accounts/views.py
+++ b/warehouse/accounts/views.py
@@ -229,6 +229,10 @@ def register(request, _form_class=RegistrationForm):
if request.authenticated_userid is not None:
return HTTPSeeOther(request.route_path('manage.projects'))
+ # Check if the honeypot field has been filled
+ if request.method == "POST" and request.POST.get('confirm_form'):
+ return HTTPSeeOther(request.route_path("index"))
+
if AdminFlag.is_enabled(request.db, 'disallow-new-user-registration'):
request.session.flash(
("New User Registration Temporarily Disabled "
@@ -238,20 +242,8 @@ def register(request, _form_class=RegistrationForm):
return HTTPSeeOther(request.route_path("index"))
user_service = request.find_service(IUserService, context=None)
- recaptcha_service = request.find_service(name="recaptcha")
- request.find_service(name="csp").merge(recaptcha_service.csp_policy)
-
- # the form contains an auto-generated field from recaptcha with
- # hyphens in it. make it play nice with wtforms.
- post_body = {
- key.replace("-", "_"): value
- for key, value in request.POST.items()
- }
- form = _form_class(
- data=post_body, user_service=user_service,
- recaptcha_service=recaptcha_service
- )
+ form = _form_class(data=request.POST, user_service=user_service,)
if request.method == "POST" and form.validate():
user = user_service.create_user(
diff --git a/warehouse/config.py b/warehouse/config.py
--- a/warehouse/config.py
+++ b/warehouse/config.py
@@ -157,8 +157,6 @@ def configure(settings=None):
maybe_set(settings, "sentry.transport", "SENTRY_TRANSPORT")
maybe_set(settings, "sessions.url", "REDIS_URL")
maybe_set(settings, "ratelimit.url", "REDIS_URL")
- maybe_set(settings, "recaptcha.site_key", "RECAPTCHA_SITE_KEY")
- maybe_set(settings, "recaptcha.secret_key", "RECAPTCHA_SECRET_KEY")
maybe_set(settings, "sessions.secret", "SESSION_SECRET")
maybe_set(settings, "camo.url", "CAMO_URL")
maybe_set(settings, "camo.key", "CAMO_KEY")
@@ -463,9 +461,6 @@ def configure(settings=None):
# Register Referrer-Policy service
config.include(".referrer_policy")
- # Register recaptcha service
- config.include(".recaptcha")
-
config.add_settings({
"http": {
"verify": "/etc/ssl/certs/",
diff --git a/warehouse/recaptcha.py b/warehouse/recaptcha.py
deleted file mode 100644
--- a/warehouse/recaptcha.py
+++ /dev/null
@@ -1,160 +0,0 @@
-# Licensed under the Apache License, Version 2.0 (the "License");
-# you may not use this file except in compliance with the License.
-# You may obtain a copy of the License at
-#
-# http://www.apache.org/licenses/LICENSE-2.0
-#
-# Unless required by applicable law or agreed to in writing, software
-# distributed under the License is distributed on an "AS IS" BASIS,
-# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-# See the License for the specific language governing permissions and
-# limitations under the License.
-
-import collections
-import http
-
-from urllib.parse import urlencode
-
-
-VERIFY_URL = "https://www.google.com/recaptcha/api/siteverify"
-
-
-class RecaptchaError(ValueError):
- pass
-
-
-class MissingInputSecretError(RecaptchaError):
- pass
-
-
-class InvalidInputSecretError(RecaptchaError):
- pass
-
-
-class MissingInputResponseError(RecaptchaError):
- pass
-
-
-class InvalidInputResponseError(RecaptchaError):
- pass
-
-
-class UnexpectedError(RecaptchaError):
- pass
-
-
-ERROR_CODE_MAP = {
- "missing-input-secret": MissingInputSecretError,
- "invalid-input-secret": InvalidInputSecretError,
- "missing-input-response": MissingInputResponseError,
- "invalid-input-response": InvalidInputResponseError,
-}
-
-ChallengeResponse = collections.namedtuple(
- "ChallengeResponse", ("challenge_ts", "hostname")
-)
-
-
-class Service:
- def __init__(self, request):
- self.request = request
-
- @property
- def csp_policy(self):
- # the use of request.scheme should ever only be for dev. problem is
- # that we use "//" in the script tags, so the request scheme is used.
- # because the csp has to match the script src scheme, it also has to
- # be dynamic.
- return {
- "script-src": [
- "{request.scheme}://www.google.com/recaptcha/",
- "{request.scheme}://www.gstatic.com/recaptcha/",
- ],
- "frame-src": [
- "{request.scheme}://www.google.com/recaptcha/",
- ],
- "style-src": [
- "'unsafe-inline'",
- ],
- }
-
- @property
- def enabled(self):
- settings = self.request.registry.settings
- return bool(settings.get("recaptcha.site_key") and
- settings.get("recaptcha.secret_key"))
-
- def verify_response(self, response, remote_ip=None):
- if not self.enabled:
- # TODO: debug logging
- return
-
- settings = self.request.registry.settings
-
- payload = {
- "secret": settings["recaptcha.secret_key"],
- "response": response,
- }
- if remote_ip is not None:
- payload["remoteip"] = remote_ip
-
- try:
- # TODO: the timeout is hardcoded for now. it would be nice to do
- # something a little more generalized in the future.
- resp = self.request.http.post(
- VERIFY_URL, urlencode(payload),
- headers={"Content-Type":
- "application/x-www-form-urlencoded; charset=utf-8"},
- timeout=10
- )
- except Exception as err:
- raise UnexpectedError(str(err))
-
- try:
- data = resp.json()
- except ValueError:
- raise UnexpectedError(
- "Unexpected data in response body: %s" % str(
- resp.content, 'utf-8')
- )
-
- if "success" not in data:
- raise UnexpectedError(
- "Missing 'success' key in response: %s" % data
- )
-
- if resp.status_code != http.HTTPStatus.OK or not data["success"]:
- try:
- error_codes = data["error_codes"]
- except KeyError:
- raise UnexpectedError(
- "Response missing 'error-codes' key: %s" % data
- )
- try:
- exc_tp = ERROR_CODE_MAP[error_codes[0]]
- except KeyError:
- raise UnexpectedError(
- "Unexpected error code: %s" % error_codes[0]
- )
- raise exc_tp
-
- # challenge_ts = timestamp of the challenge load
- # (ISO format yyyy-MM-dd'T'HH:mm:ssZZ)
- # TODO: maybe run some validation against the hostname and timestamp?
- # TODO: log if either field is empty.. it shouldn't cause a failure,
- # but it likely means that google has changed their response structure
- return ChallengeResponse(
- data.get("challenge_ts"),
- data.get("hostname"),
- )
-
-
-def service_factory(handler, request):
- return Service(request)
-
-
-def includeme(config):
- # yeah yeah, binding to a concrete implementation rather than an
- # interface. in a perfect world, this will never be offloaded to another
- # service. however, if it is, then we'll deal with the refactor then
- config.register_service_factory(service_factory, name="recaptcha")
| diff --git a/tests/unit/accounts/test_forms.py b/tests/unit/accounts/test_forms.py
--- a/tests/unit/accounts/test_forms.py
+++ b/tests/unit/accounts/test_forms.py
@@ -16,7 +16,6 @@
from warehouse.accounts import forms
from warehouse.accounts.interfaces import TooManyFailedLogins
-from warehouse import recaptcha
class TestLoginForm:
@@ -127,14 +126,9 @@ class TestRegistrationForm:
def test_create(self):
user_service = pretend.stub()
- recaptcha_service = pretend.stub(enabled=True)
- form = forms.RegistrationForm(
- data={}, user_service=user_service,
- recaptcha_service=recaptcha_service
- )
+ form = forms.RegistrationForm(data={}, user_service=user_service)
assert form.user_service is user_service
- assert form.recaptcha_service is recaptcha_service
def test_password_confirm_required_error(self):
form = forms.RegistrationForm(
@@ -144,7 +138,6 @@ def test_password_confirm_required_error(self):
lambda _: pretend.stub()
),
),
- recaptcha_service=pretend.stub(enabled=True),
)
assert not form.validate()
@@ -162,7 +155,6 @@ def test_passwords_mismatch_error(self):
"password_confirm": "mismatch",
},
user_service=user_service,
- recaptcha_service=pretend.stub(enabled=True),
)
assert not form.validate()
@@ -183,7 +175,6 @@ def test_passwords_match_success(self):
"password_confirm": "MyStr0ng!shPassword",
},
user_service=user_service,
- recaptcha_service=pretend.stub(enabled=True),
)
form.validate()
@@ -198,7 +189,6 @@ def test_email_required_error(self):
lambda _: pretend.stub()
),
),
- recaptcha_service=pretend.stub(enabled=True),
)
assert not form.validate()
@@ -210,7 +200,6 @@ def test_invalid_email_error(self):
user_service=pretend.stub(
find_userid_by_email=pretend.call_recorder(lambda _: None),
),
- recaptcha_service=pretend.stub(enabled=True),
)
assert not form.validate()
@@ -228,7 +217,6 @@ def test_email_exists_error(self):
lambda _: pretend.stub()
),
),
- recaptcha_service=pretend.stub(enabled=True),
)
assert not form.validate()
@@ -244,7 +232,6 @@ def test_blacklisted_email_error(self):
user_service=pretend.stub(
find_userid_by_email=pretend.call_recorder(lambda _: None),
),
- recaptcha_service=pretend.stub(enabled=True),
)
assert not form.validate()
@@ -254,56 +241,12 @@ def test_blacklisted_email_error(self):
"this domain. Please use a different email."
)
- def test_recaptcha_disabled(self):
- form = forms.RegistrationForm(
- data={"g_recpatcha_response": ""},
- user_service=pretend.stub(),
- recaptcha_service=pretend.stub(
- enabled=False,
- verify_response=pretend.call_recorder(lambda _: None),
- ),
- )
- assert not form.validate()
- # there shouldn't be any errors for the recaptcha field if it's
- # disabled
- assert not form.g_recaptcha_response.errors
-
- def test_recaptcha_required_error(self):
- form = forms.RegistrationForm(
- data={"g_recaptcha_response": ""},
- user_service=pretend.stub(),
- recaptcha_service=pretend.stub(
- enabled=True,
- verify_response=pretend.call_recorder(lambda _: None),
- ),
- )
- assert not form.validate()
- assert form.g_recaptcha_response.errors.pop() \
- == "Recaptcha error."
-
- def test_recaptcha_error(self):
- form = forms.RegistrationForm(
- data={"g_recaptcha_response": "asd"},
- user_service=pretend.stub(),
- recaptcha_service=pretend.stub(
- verify_response=pretend.raiser(recaptcha.RecaptchaError),
- enabled=True,
- ),
- )
- assert not form.validate()
- assert form.g_recaptcha_response.errors.pop() \
- == "Recaptcha error."
-
def test_username_exists(self):
form = forms.RegistrationForm(
data={"username": "foo"},
user_service=pretend.stub(
find_userid=pretend.call_recorder(lambda name: 1),
),
- recaptcha_service=pretend.stub(
- enabled=False,
- verify_response=pretend.call_recorder(lambda _: None),
- ),
)
assert not form.validate()
assert (
@@ -319,10 +262,6 @@ def test_username_is_valid(self, username):
user_service=pretend.stub(
find_userid=pretend.call_recorder(lambda _: None),
),
- recaptcha_service=pretend.stub(
- enabled=False,
- verify_response=pretend.call_recorder(lambda _: None),
- ),
)
assert not form.validate()
assert (
@@ -344,10 +283,6 @@ def test_password_strength(self):
form = forms.RegistrationForm(
data={"new_password": pwd, "password_confirm": pwd},
user_service=pretend.stub(),
- recaptcha_service=pretend.stub(
- enabled=False,
- verify_response=pretend.call_recorder(lambda _: None),
- ),
)
form.validate()
assert (len(form.new_password.errors) == 0) == valid
diff --git a/tests/unit/accounts/test_views.py b/tests/unit/accounts/test_views.py
--- a/tests/unit/accounts/test_views.py
+++ b/tests/unit/accounts/test_views.py
@@ -333,6 +333,23 @@ def test_redirect_authenticated_user(self):
assert isinstance(result, HTTPSeeOther)
assert result.headers["Location"] == "/the-redirect"
+ def test_register_honeypot(self, pyramid_request, monkeypatch):
+ pyramid_request.method = "POST"
+ create_user = pretend.call_recorder(lambda *args, **kwargs: None)
+ add_email = pretend.call_recorder(lambda *args, **kwargs: None)
+ pyramid_request.route_path = pretend.call_recorder(lambda name: "/")
+ pyramid_request.POST = {'confirm_form': '[email protected]'}
+ send_email = pretend.call_recorder(lambda *a: None)
+ monkeypatch.setattr(views, 'send_email_verification_email', send_email)
+
+ result = views.register(pyramid_request)
+
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/"
+ assert create_user.calls == []
+ assert add_email.calls == []
+ assert send_email.calls == []
+
def test_register_redirect(self, db_request, monkeypatch):
db_request.method = "POST"
diff --git a/tests/unit/test_config.py b/tests/unit/test_config.py
--- a/tests/unit/test_config.py
+++ b/tests/unit/test_config.py
@@ -347,7 +347,6 @@ def __init__(self):
pretend.call(".raven"),
pretend.call(".csp"),
pretend.call(".referrer_policy"),
- pretend.call(".recaptcha"),
pretend.call(".http"),
] + [
pretend.call(x) for x in [
diff --git a/tests/unit/test_recaptcha.py b/tests/unit/test_recaptcha.py
deleted file mode 100644
--- a/tests/unit/test_recaptcha.py
+++ /dev/null
@@ -1,268 +0,0 @@
-# Licensed under the Apache License, Version 2.0 (the "License");
-# you may not use this file except in compliance with the License.
-# You may obtain a copy of the License at
-#
-# http://www.apache.org/licenses/LICENSE-2.0
-#
-# Unless required by applicable law or agreed to in writing, software
-# distributed under the License is distributed on an "AS IS" BASIS,
-# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-# See the License for the specific language governing permissions and
-# limitations under the License.
-
-import socket
-import urllib.parse
-
-import pytest
-import pretend
-import requests
-import responses
-
-from warehouse import recaptcha
-
-
-_SETTINGS = {
- "recaptcha.site_key": "site_key_value",
- "recaptcha.secret_key": "secret_key_value",
-}
-_REQUEST = pretend.stub(
- # returning a real requests.Session object because responses is responsible
- # for mocking that out
- http=requests.Session(),
- registry=pretend.stub(
- settings=_SETTINGS,
- ),
-)
-
-
-class TestVerifyResponse:
- @responses.activate
- def test_verify_service_disabled(self):
- responses.add(
- responses.POST,
- recaptcha.VERIFY_URL,
- body="",
- )
- serv = recaptcha.Service(
- pretend.stub(registry=pretend.stub(settings={}))
- )
- assert serv.verify_response('') is None
- assert not responses.calls
-
- @responses.activate
- def test_verify_service_disabled_with_none(self):
- responses.add(
- responses.POST,
- recaptcha.VERIFY_URL,
- body="",
- )
- serv = recaptcha.Service(
- pretend.stub(
- registry=pretend.stub(
- settings={
- "recaptcha.site_key": None,
- "recaptcha.secret_key": None,
- },
- ),
- ),
- )
- assert serv.verify_response('') is None
- assert not responses.calls
-
- @responses.activate
- def test_remote_ip_payload(self):
- responses.add(
- responses.POST,
- recaptcha.VERIFY_URL,
- json={"success": True},
- )
- serv = recaptcha.Service(_REQUEST)
- serv.verify_response("meaningless", remote_ip="ip")
-
- payload = dict(urllib.parse.parse_qsl(responses.calls[0].request.body))
- assert payload["remoteip"] == "ip"
-
- @responses.activate
- def test_unexpected_data_error(self):
- responses.add(
- responses.POST,
- recaptcha.VERIFY_URL,
- body="something awful",
- )
- serv = recaptcha.Service(_REQUEST)
-
- with pytest.raises(recaptcha.UnexpectedError) as err:
- serv.verify_response("meaningless")
-
- expected = "Unexpected data in response body: something awful"
- assert str(err.value) == expected
-
- @responses.activate
- def test_missing_success_key_error(self):
- responses.add(
- responses.POST,
- recaptcha.VERIFY_URL,
- json={"foo": "bar"},
- )
- serv = recaptcha.Service(_REQUEST)
-
- with pytest.raises(recaptcha.UnexpectedError) as err:
- serv.verify_response("meaningless")
-
- expected = "Missing 'success' key in response: {'foo': 'bar'}"
- assert str(err.value) == expected
-
- @responses.activate
- def test_missing_error_codes_key_error(self):
- responses.add(
- responses.POST,
- recaptcha.VERIFY_URL,
- json={"success": False},
- )
- serv = recaptcha.Service(_REQUEST)
-
- with pytest.raises(recaptcha.UnexpectedError) as err:
- serv.verify_response("meaningless")
-
- expected = "Response missing 'error-codes' key: {'success': False}"
- assert str(err.value) == expected
-
- @responses.activate
- def test_error_map_error(self):
- for key, exc_tp in recaptcha.ERROR_CODE_MAP.items():
- responses.add(
- responses.POST,
- recaptcha.VERIFY_URL,
- json={
- "success": False,
- "challenge_ts": 0,
- "hostname": "hotname_value",
- "error_codes": [key]
- }
- )
-
- serv = recaptcha.Service(_REQUEST)
- with pytest.raises(exc_tp):
- serv.verify_response("meaningless")
-
- responses.reset()
-
- @responses.activate
- def test_error_map_unknown_error(self):
- responses.add(
- responses.POST,
- recaptcha.VERIFY_URL,
- json={
- "success": False,
- "challenge_ts": 0,
- "hostname": "hostname_value",
- "error_codes": ["slartibartfast"],
- },
- )
-
- serv = recaptcha.Service(_REQUEST)
- with pytest.raises(recaptcha.UnexpectedError) as err:
- serv.verify_response("meaningless")
- assert str(err) == "Unexpected error code: slartibartfast"
-
- @responses.activate
- def test_challenge_response_missing_timestamp_success(self):
- responses.add(
- responses.POST,
- recaptcha.VERIFY_URL,
- json={
- "success": True,
- "hostname": "hostname_value",
- },
- )
-
- serv = recaptcha.Service(_REQUEST)
- res = serv.verify_response("meaningless")
-
- assert isinstance(res, recaptcha.ChallengeResponse)
- assert res.challenge_ts is None
- assert res.hostname == "hostname_value"
-
- @responses.activate
- def test_challenge_response_missing_hostname_success(self):
- responses.add(
- responses.POST,
- recaptcha.VERIFY_URL,
- json={
- "success": True,
- "challenge_ts": 0,
- },
- )
-
- serv = recaptcha.Service(_REQUEST)
- res = serv.verify_response("meaningless")
-
- assert isinstance(res, recaptcha.ChallengeResponse)
- assert res.hostname is None
- assert res.challenge_ts == 0
-
- @responses.activate
- def test_challenge_response_success(self):
- responses.add(
- responses.POST,
- recaptcha.VERIFY_URL,
- json={
- "success": True,
- "hostname": "hostname_value",
- "challenge_ts": 0,
- },
- )
-
- serv = recaptcha.Service(_REQUEST)
- res = serv.verify_response("meaningless")
-
- assert isinstance(res, recaptcha.ChallengeResponse)
- assert res.hostname == "hostname_value"
- assert res.challenge_ts == 0
-
- @responses.activate
- def test_unexpected_error(self):
- serv = recaptcha.Service(_REQUEST)
- serv.request.http.post = pretend.raiser(socket.error)
-
- with pytest.raises(recaptcha.UnexpectedError):
- serv.verify_response("meaningless")
-
-
-class TestCSPPolicy:
- def test_csp_policy(self):
- scheme = 'https'
- request = pretend.stub(
- scheme=scheme,
- registry=pretend.stub(settings={
- "recaptcha.site_key": "foo",
- "recaptcha.secret_key": "bar",
- })
- )
- serv = recaptcha.Service(request)
- assert serv.csp_policy == {
- "script-src": [
- "{request.scheme}://www.google.com/recaptcha/",
- "{request.scheme}://www.gstatic.com/recaptcha/",
- ],
- "frame-src": ["{request.scheme}://www.google.com/recaptcha/"],
- "style-src": ["'unsafe-inline'"],
- }
-
-
-def test_service_factory():
- serv = recaptcha.service_factory(None, _REQUEST)
- assert serv.request is _REQUEST
-
-
-def test_includeme():
- config = pretend.stub(
- register_service_factory=pretend.call_recorder(
- lambda fact, name: None
- ),
- )
- recaptcha.includeme(config)
-
- assert config.register_service_factory.calls == [
- pretend.call(recaptcha.service_factory, name="recaptcha"),
- ]
| reCAPTCHA blocked in China
A pypi ID is necessary while Iwould like to share and upload a package on pypi. Unfortunnatly, there was something wrong with the website of "https://pypi.org/account/register/". After writing the all information, an ERROR was arise named "Recaptcha error.".
I'm not sure if that is caused by China Internet?
| It looks like our Register page may be blocking the "additional screening" required by the recaptcha on occasion. Generally a modal that pops over and requests that a user click on objects that look like a "street sign" or "storefront"
@di @nlhkabu any ideas?
Might be something related to the CSP policy.
@JacksonWuxs Are you seeing a reCAPTCHA like this on the register page?
<img width="479" alt="screen shot 2018-03-08 at 9 15 06 am" src="https://user-images.githubusercontent.com/294415/37158553-419d92b2-22b1-11e8-8ecb-5c0507a7afc9.png">
@di @ewdurbin I also don't see CAPTCHA in China. See below,


Oh interesting, I wonder if China is blocking recaptcha.
Okay, so according to @reaperhulk Recaptcha doesn't work in China, that is... unfortunate.
Some more information https://github.com/google/recaptcha/issues/87
@dstufft I see, thanks.
Not sure what (if any) milestone we should add this to, but getting this fixed is super important since currently all of China can't interact with anything on Warehouse that requires a captcha. /cc @brainwane
I'm sorry about writing back so late.
In fact I didn't see this register page. Here is what I got after clicking the "Create Account" button.
I will try to find the answer from the blog what you gave me. In any case, I am moved by your attention to this issue.
By the way, Is the user who upload the library to Pypi really important? If not, could you help me upload my lib to Pypi please? I can't wait to share my library. hahaha...
Here is my lib address: https://github.com/JacksonWuxs/datapy .The address includes CODES, README and EXAMPLES.
If you need more information about this issue, please let me know. I will try my best to collect information to help you.
@JacksonWuxs If you let me know the email address and username you'd like to use, I can register an account on your behalf, and then we can do a password reset so you can get access to it.
I'm really happy to hear that. I hope you could help me register an account and my information as follow:
E-Mail:[email protected]
User Name: Jackson Woo
Real Name: Xuansheng Wu
@JacksonWuxs Done, you should have a password reset email in your inbox.
Leaving this issue open until we resolve the larger problem of using reCAPTCHA in China.
Done, I have finished to reset my password. Thanks, so much!
I'm sorry I need to bother you again. Unfortunately, when I was uploading my program with command as "twine upload dist/*", I raised another error. The error said: "HTTPError: 403 Client Error: The user 'JacksonWoo' is not allowed to upload to project 'datapy'."
How can I do?
@JacksonWuxs No apology necessary. [The `datapy` project already exists](https://pypi.org/project/datapy/), you'll need to choose a new name.
Oh it so sad... Could you tell me which web site will show the name list?
I mean the token names.
@JacksonWuxs You can search here: https://pypi.org/search/, for example https://pypi.org/search/?q=datapy.
I upload my package to Pypi successful.
But I faced another bigger problem I thought. After I install successful, it still couldn't be import? I have screenshot of the wrong process. Thank you!
On 3/12/2018 22:02,Dustin Ingram<[email protected]> wrote:
@JacksonWuxs No apology necessary. The datapy project already exists, you'll need to choose a new name.
—
You are receiving this because you were mentioned.
Reply to this email directly, view it on GitHub, or mute the thread.
I'd love advice on how we should address this. Right now I believe this is the biggest blocker stopping us from going to the beta stage and doing our publicity push.
We're probably going to have to use a different captcha solution, at least in china if not everywhere. At least that's the only thing I can think of.
Some basic notes at https://stackoverflow.com/questions/23780387/recaptcha-availability-in-china that sum up to "Don't use recaptcha if you have users in China".
It's been a while but I've used this with success when I needed a captcha solution that worked in China in the past: https://captcha.com/ (note that there's no Python backend though.)
@brainwane asked me for input on this, so I read the entire upstream issue (google/recaptcha#87). In summary:
- reCAPTCHA was reported blocked in China in January 2016. I believe this is because parts of it are hosted under `https://www.google.com/recaptcha/`, and since SSL encrypts the URL being requested, this is indistinguishable from any other request to `www.google.com` from the Great Firewall's perspective
- There's a lot of confusing discussion and back-and-forth where people try to figure out a solution that proxies reCAPTCHA on their domain. This is harder than it sounds because reCAPTCHA serves JS that makes further requests to reCAPTCHA, so a proxy has to rewrite URLs embedded in the responses to point to itself instead of to Google.
- Proxy solutions that people claim work for them are [this one for nginx](https://github.com/google/recaptcha/issues/87#issuecomment-371612248), and either [this one](https://github.com/google/recaptcha/issues/87#issuecomment-353927114), or [this one](https://github.com/google/recaptcha/issues/87#issuecomment-276258765) with [this addendum](https://github.com/google/recaptcha/issues/87#issuecomment-360925035), for Apache. However, the latter is reported to have [some issues](https://github.com/google/recaptcha/issues/87#issuecomment-361036541), and I wouldn't be surprised if other proxy solutions had similar problems. Rewriting a third party's JS on the fly to get it to send all its requests to your domain is tricky and fragile.
- Someone [claimed](https://github.com/google/recaptcha/issues/87#issuecomment-368252094) that https://recaptcha.net would work, because that domain is [not blocked](https://en.greatfire.org/https/recaptcha.net) according to greatfire.org, and its SSL certificate is Google's misc services cert (so it looks legit). However, [someone else reported](https://github.com/google/recaptcha/issues/87#issuecomment-371610398) that recaptcha.net still makes a request to `www.google.com`, which would fail in China.
My recommendation would be to first try to get Google to fix their own proxy (recaptcha.net) first, since it appears to almost work (if it is indeed broken; that's probably worth independently verifying). If that fails, you could try setting up your own proxy, but that is likely to be buggy and require frequent maintenance, since routine changes made by Google to the reCAPTCHA code are likely to break the content rewrite rules from time to time. When such a proxy does break, it could break captcha functionality for all users, not just those in China. As an alternative, it might be less trouble to use a different captcha service, as @tylerdave suggests.
Assuming the purpose of a CAPTCHA is to prevent malicious users from registering accounts, there are other proven techniques that do not require it in any form. I've successfully implemented many of the techniques described in this article:
https://webaim.org/blog/spam_free_accessible_forms/
These techniques are transparent to the legitimate user (no extra click, selection of specific objects in squares, or transcription of wiggly alphanumerics), are accessible (this is extremely important), and they effectively eliminate most automated attacks.
Please consider this strategy instead of *CAPTCHA. Thank you!
It's very unfortunate to see this. IMO we shouldn't rely on any work-around on reCAPTCHA itself, you don't know China's policy when it comes to google. Trust me.
Looking at https://webaim.org/blog/spam_free_accessible_forms/ I'm not sure those techniques are going to help us with registration spam:
> Detect spam-like content within submitted form elements
There is not really enough content on the submitted fields to do this
> Detect content within a hidden form element
I don't think this is applicable here.
> Validate the submitted form values
Already do this, and blacklist disposable email domains.
> Search for the same content in multiple form elements
I don't think this is applicable here.
> Generate dynamic content to ensure the form is submitted within a specific time window or by the same user
Already do this with CSRF tokens.
> Create a multi-stage form or form verification page
I don't think we want to do this for our registration form.
> Ensure the form is posted from your server
Checking the referrer is something we can do, but this is easy to forge.
I think I'm going to try switching to https://captcha.com/, it's both available in China and touts it's accessibility so it shouldn't be much different than reCAPTCHA for our users.
Hmm, it seems like https://captcha.com/ does not offer a hosted solution (probably why it works so well in China) so the lack of a Python backend will be a problem, and requires a license.
I hope y'all decide to abandon CAPTCHAs. If so, then I'd ask that you reconsider these three tactics. They have worked very well for me, second only to rigorous submitted value validation and CSRF protection, to stop automated evildoers.
* Detect spam-like content within submitted form elements
* Detect content within a hidden form element # This "honeypot" catches many spambots for me
* Search for the same content in multiple form elements
Of course, stopping attacks further upstream would be even better, but that's above my pay grade and for systems and network administrators. 😉 | 2018-03-21T21:40:23Z | [] | [] |
pypi/warehouse | 3,352 | pypi__warehouse-3352 | [
"3336"
] | a8d04e0aea2eae04b6705fdcadfbcb1712ecc214 | diff --git a/warehouse/cache/origin/__init__.py b/warehouse/cache/origin/__init__.py
--- a/warehouse/cache/origin/__init__.py
+++ b/warehouse/cache/origin/__init__.py
@@ -15,6 +15,8 @@
import operator
from itertools import chain
+from sqlalchemy.orm.session import Session
+
from warehouse import db
from warehouse.cache.origin.interfaces import IOriginCache
@@ -132,6 +134,19 @@ def register_origin_cache_keys(config, klass, cache_keys=None,
)
+def receive_set(attribute, config, target):
+ cache_keys = config.registry["cache_keys"]
+ session = Session.object_session(target)
+ if session:
+ purges = session.info.setdefault(
+ "warehouse.cache.origin.purges",
+ set()
+ )
+ key_maker = cache_keys[attribute]
+ keys = key_maker(target).purge
+ purges.update(list(keys))
+
+
def includeme(config):
if "origin_cache.backend" in config.registry.settings:
cache_class = config.maybe_dotted(
diff --git a/warehouse/packaging/__init__.py b/warehouse/packaging/__init__.py
--- a/warehouse/packaging/__init__.py
+++ b/warehouse/packaging/__init__.py
@@ -11,14 +11,25 @@
# limitations under the License.
from celery.schedules import crontab
+from warehouse import db
-from warehouse.accounts.models import User
-from warehouse.cache.origin import key_factory
+from warehouse.accounts.models import User, Email
+from warehouse.cache.origin import key_factory, receive_set
from warehouse.packaging.interfaces import IFileStorage
from warehouse.packaging.models import Project, Release
from warehouse.packaging.tasks import compute_trending
[email protected]_for(User.name, 'set')
+def user_name_receive_set(config, target, value, oldvalue, initiator):
+ receive_set(User.name, config, target)
+
+
[email protected]_for(Email.primary, 'set')
+def email_primary_receive_set(config, target, value, oldvalue, initiator):
+ receive_set(Email.primary, config, target)
+
+
def includeme(config):
# Register whatever file storage backend has been configured for storing
# our package files.
@@ -49,11 +60,24 @@ def includeme(config):
config.register_origin_cache_keys(
User,
cache_keys=["user/{obj.username}"],
+ )
+ config.register_origin_cache_keys(
+ User.name,
purge_keys=[
key_factory("user/{obj.username}"),
key_factory("project/{itr.normalized_name}", iterate_on='projects')
],
)
+ config.register_origin_cache_keys(
+ Email.primary,
+ purge_keys=[
+ key_factory("user/{obj.user.username}"),
+ key_factory(
+ "project/{itr.normalized_name}",
+ iterate_on='user.projects',
+ )
+ ],
+ )
# Add a periodic task to compute trending once a day, assuming we have
# been configured to be able to access BigQuery.
| diff --git a/tests/common/db/accounts.py b/tests/common/db/accounts.py
--- a/tests/common/db/accounts.py
+++ b/tests/common/db/accounts.py
@@ -37,6 +37,7 @@ class Meta:
last_login = factory.fuzzy.FuzzyNaiveDateTime(
datetime.datetime(2011, 1, 1),
)
+ projects = []
class EmailFactory(WarehouseFactory):
diff --git a/tests/unit/packaging/test_init.py b/tests/unit/packaging/test_init.py
--- a/tests/unit/packaging/test_init.py
+++ b/tests/unit/packaging/test_init.py
@@ -16,8 +16,9 @@
from celery.schedules import crontab
from warehouse import packaging
+from warehouse.accounts.models import Email, User
from warehouse.packaging.interfaces import IFileStorage
-from warehouse.packaging.models import Project, Release, User
+from warehouse.packaging.models import Project, Release
from warehouse.packaging.tasks import compute_trending
@@ -73,6 +74,9 @@ def key_factory(keystring, iterate_on=None):
pretend.call(
User,
cache_keys=["user/{obj.username}"],
+ ),
+ pretend.call(
+ User.name,
purge_keys=[
key_factory("user/{obj.username}"),
key_factory(
@@ -81,6 +85,16 @@ def key_factory(keystring, iterate_on=None):
),
],
),
+ pretend.call(
+ Email.primary,
+ purge_keys=[
+ key_factory("user/{obj.user.username}"),
+ key_factory(
+ "project/{itr.normalized_name}",
+ iterate_on='user.projects',
+ ),
+ ],
+ ),
]
if with_trending:
| Unnecessary purges on `User` model change
Currently right now any time the `User` model changes, we purge all the cache keys for that user's project.
This includes attribute changes that don't actually affect the project pages, like `last_login`, `password` etc.
We should filter out "purge-able" attribute changes and only issue purges when necessary. Said attributes include:
* `username`
* `name`
* `emails`
| ~~it also issues an `all-projects` which purges `/simple`~~
\* was looking at wrong log :)
Hmm, shouldn't be...
my bad, sneaky edited my original comment after checking logs more closely.
Ernest mentioned we need this before launching beta, because otherwise it's so costly to serve users who have many projects each. | 2018-03-22T12:34:39Z | [] | [] |
pypi/warehouse | 3,378 | pypi__warehouse-3378 | [
"3163"
] | 02836f0edbcfc357e29661deb0ea759fa7004eb1 | diff --git a/warehouse/manage/views.py b/warehouse/manage/views.py
--- a/warehouse/manage/views.py
+++ b/warehouse/manage/views.py
@@ -473,7 +473,7 @@ def delete_project_release(self):
@view_config(
request_method="POST",
- request_param=["confirm_filename", "file_id"]
+ request_param=["confirm_project_name", "file_id"]
)
def delete_project_release_file(self):
@@ -487,9 +487,9 @@ def _error(message):
)
)
- filename = self.request.POST.get('confirm_filename')
+ project_name = self.request.POST.get('confirm_project_name')
- if not filename:
+ if not project_name:
return _error("Must confirm the request.")
try:
@@ -504,10 +504,11 @@ def _error(message):
except NoResultFound:
return _error('Could not find file.')
- if filename != release_file.filename:
+ if project_name != self.release.project.name:
return _error(
"Could not delete file - " +
- f"{filename!r} is not the same as {release_file.filename!r}",
+ f"{project_name!r} is not the same as "
+ f"{self.release.project.name!r}",
)
self.request.db.add(
| diff --git a/tests/unit/manage/test_views.py b/tests/unit/manage/test_views.py
--- a/tests/unit/manage/test_views.py
+++ b/tests/unit/manage/test_views.py
@@ -1096,7 +1096,7 @@ def test_delete_project_release_file(self, monkeypatch):
)
request = pretend.stub(
POST={
- 'confirm_filename': release_file.filename,
+ 'confirm_project_name': release.project.name,
'file_id': release_file.id,
},
method="POST",
@@ -1156,7 +1156,7 @@ def test_delete_project_release_file_no_confirm(self):
project=pretend.stub(name='foobar'),
)
request = pretend.stub(
- POST={'confirm_filename': ''},
+ POST={'confirm_project_name': ''},
method="POST",
db=pretend.stub(delete=pretend.call_recorder(lambda a: None)),
route_path=pretend.call_recorder(lambda *a, **kw: '/the-redirect'),
@@ -1195,7 +1195,7 @@ def no_result_found():
raise NoResultFound
request = pretend.stub(
- POST={'confirm_filename': 'whatever'},
+ POST={'confirm_project_name': 'whatever'},
method="POST",
db=pretend.stub(
delete=pretend.call_recorder(lambda a: None),
@@ -1239,7 +1239,7 @@ def test_delete_project_release_file_bad_confirm(self):
project=pretend.stub(name='foobar'),
)
request = pretend.stub(
- POST={'confirm_filename': 'invalid'},
+ POST={'confirm_project_name': 'invalid'},
method="POST",
db=pretend.stub(
delete=pretend.call_recorder(lambda a: None),
@@ -1263,7 +1263,7 @@ def test_delete_project_release_file_bad_confirm(self):
assert request.session.flash.calls == [
pretend.call(
"Could not delete file - " +
- f"'invalid' is not the same as {release_file.filename!r}",
+ f"'invalid' is not the same as {release.project.name!r}",
queue="error",
)
]
| Change delete file modal confirmation process
**From user testing:**
Currently, to delete a file, you must confirm the entire file name:
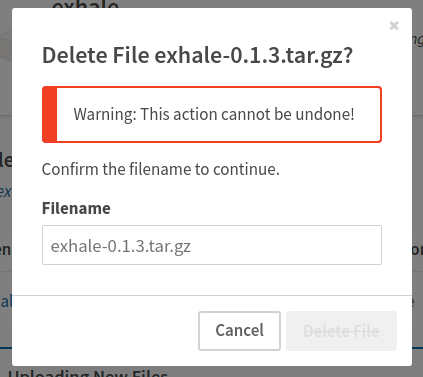
This is a little bit painful for some users, because the file name can get pretty long.
Instead, we should change this modal so that we require the `project name` for confirmation.
---
**Good First Issue**: This issue is good for first time contributors. If you've already contributed to Warehouse, please work on [another issue without this label](https://github.com/pypa/warehouse/issues?utf8=%E2%9C%93&q=is%3Aissue+is%3Aopen+-label%3A%22good+first+issue%22) instead. If there is not a corresponding pull request for this issue, it is up for grabs. For directions for getting set up, see our [Getting Started Guide](https://warehouse.pypa.io/development/getting-started/). If you are working on this issue and have questions, please feel free to ask them here, [`#pypa-dev` on Freenode](https://webchat.freenode.net/?channels=%23pypa-dev), or the [pypa-dev mailing list](https://groups.google.com/forum/#!forum/pypa-dev).
| @aalmazan could I ask for you to help with this?
@brainwane Sure, however, I can't seem to find a good way to test uploading packages. Are there some docs you can point me to? Or at least a high-level of how I can create a test package?
Would I need to follow [the packaging guide](https://packaging.python.org/tutorials/distributing-packages/) while having pypi.org point to localhost?
@aalmazan As a first step, if you haven't uploaded a package to PyPI before, I suggest you follow [the packaging guide](https://packaging.python.org/tutorials/distributing-packages/) and then [upload your package to Test PyPI](https://packaging.python.org/guides/using-testpypi/), just to see how the whole process works. [Here's a toy project I have set up reasonably well](https://gitlab.com/brainwane/form990s) in case you want to clone and use that (you'll have to modify the package name in `setup.py` so Test PyPI will let you upload it).
I am realizing now that [our Getting Started guide](https://warehouse.readthedocs.io/development/getting-started/) lacks information on uploading packages locally. @waseem18 and @lgh2 I think you've dealt with this -- how did you manage it? Whatever you advise, we should write it up and add it to the docs!
@aalmazan Sorry for this oversight!
@aalmazan What you can do is - Find a user from local database who has one or more packages then reset the user's password and login with the same user. You can then use the user's packages to reproduce any issues.
@brainwane I'll add this information to the `Getting Started` guide sometime today.
Hi everyone, we actually already have documentation for how to login locally here: https://warehouse.readthedocs.io/development/getting-started/#logging-in-to-warehouse
@brainwane Our guide already has some details about uploading packages locally, but it's not in the "Getting Started" section: https://warehouse.pypa.io/development/reviewing-patches/?highlight=twine#testing-with-twine
I just spoke with Nicole and we agreed that this can wait till after we shut down legacy PyPI.
@aalmazan do you have what you need to proceed on this? Please do go ahead and work on it -- if we can merge your fix before shutting down legacy, all the better!
Below is a screenshot of what I currently have for the modal copy. Let me know if this should change. I think with how the `confirm_modal` macro is now, I'm not able to add more detail about what's deleted unless I add in some sort of `extra_details=[html_strings]` to the macro? Maybe we can add in the filename in the modal title if we're fine with having the title wrap to two lines? (i.e. "Delete File (filename.tar.gz) From pypusu?")
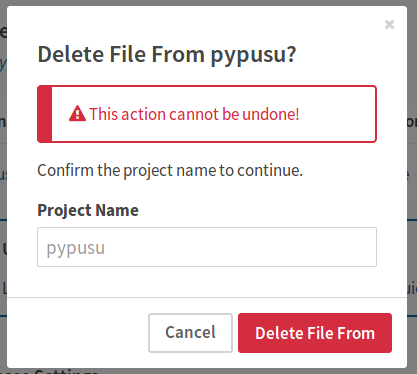
----
Additionally, there seems to be a bug with the delete modal not clearing the delete button's previous state on close (see gif below). I don't think I see this reported in issues either -- has it been reported yet? I can fix the bug as well as I work on this issue.
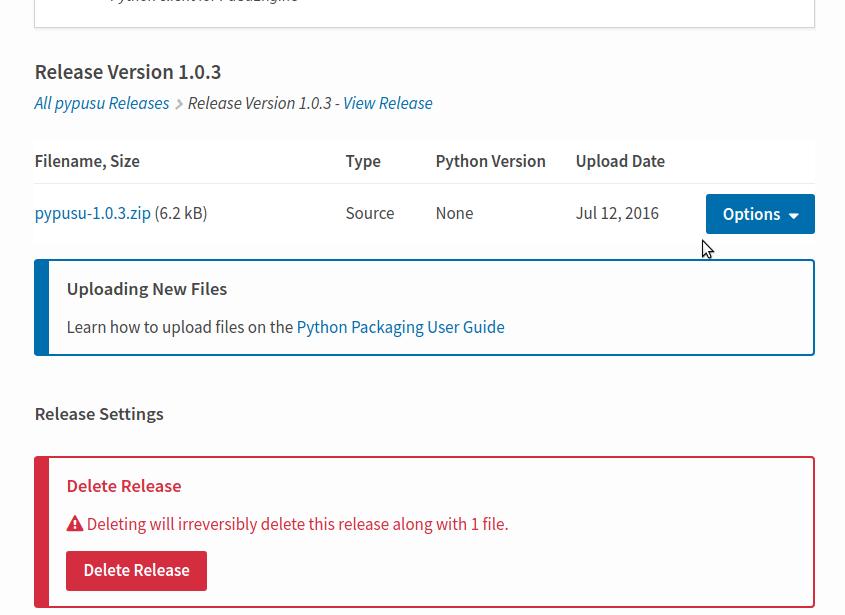
Hi @aalmazan - thanks for your work on this! I think just "delete file from <project name>" for the title is fine (we had feedback during user tests that the file name itself could be really really long).
I agree that it would be nice to make another argument in the macro - but maybe for an additional line of information in the modal. For example:
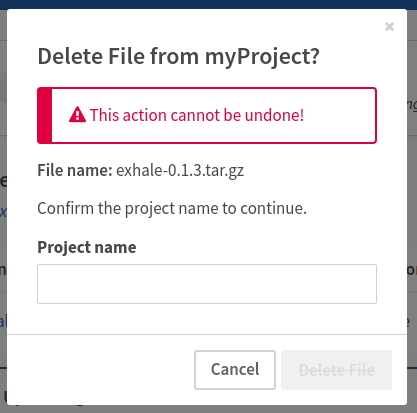
This would allow us to be more flexible with the main macro content in the future. What do you think?
---------------------
Thanks for finding the button bug as well, I'll open a new issue for this :)
| 2018-03-23T06:05:24Z | [] | [] |
pypi/warehouse | 3,393 | pypi__warehouse-3393 | [
"164"
] | 9ea202d21b960ce234fa7eb15f3c3ef7a746ebfc | diff --git a/warehouse/accounts/views.py b/warehouse/accounts/views.py
--- a/warehouse/accounts/views.py
+++ b/warehouse/accounts/views.py
@@ -35,7 +35,6 @@
send_password_reset_email, send_email_verification_email,
)
from warehouse.packaging.models import Project, Release
-from warehouse.utils.admin_flags import AdminFlag
from warehouse.utils.http import is_safe_url
@@ -233,7 +232,7 @@ def register(request, _form_class=RegistrationForm):
if request.method == "POST" and request.POST.get('confirm_form'):
return HTTPSeeOther(request.route_path("index"))
- if AdminFlag.is_enabled(request.db, 'disallow-new-user-registration'):
+ if request.flags.enabled('disallow-new-user-registration'):
request.session.flash(
("New User Registration Temporarily Disabled "
"See https://pypi.org/help#admin-intervention for details"),
diff --git a/warehouse/admin/__init__.py b/warehouse/admin/__init__.py
--- a/warehouse/admin/__init__.py
+++ b/warehouse/admin/__init__.py
@@ -10,10 +10,10 @@
# See the License for the specific language governing permissions and
# limitations under the License.
-from warehouse.accounts.views import login, logout
-
def includeme(config):
+ from warehouse.accounts.views import login, logout
+
# Setup Jinja2 Rendering for the Admin application
config.add_jinja2_search_path("templates", name=".html")
@@ -23,6 +23,9 @@ def includeme(config):
# Add our routes
config.include(".routes")
+ # Add our flags
+ config.include(".flags")
+
config.add_view(
login,
route_name="admin.login",
diff --git a/warehouse/utils/admin_flags.py b/warehouse/admin/flags.py
similarity index 53%
rename from warehouse/utils/admin_flags.py
rename to warehouse/admin/flags.py
--- a/warehouse/utils/admin_flags.py
+++ b/warehouse/admin/flags.py
@@ -10,24 +10,36 @@
# See the License for the specific language governing permissions and
# limitations under the License.
-from sqlalchemy import Column, Boolean, Text
+from sqlalchemy import Column, Boolean, Text, sql
from warehouse import db
-class AdminFlag(db.Model):
+class AdminFlag(db.ModelBase):
__tablename__ = "warehouse_admin_flag"
id = Column(Text, primary_key=True, nullable=False)
description = Column(Text, nullable=False)
enabled = Column(Boolean, nullable=False)
+ notify = Column(Boolean, nullable=False, server_default=sql.false())
- @classmethod
- def is_enabled(cls, session, flag_name):
- flag = (session.query(cls)
- .filter(cls.id == flag_name)
- .first())
- if flag is None:
- return False
- return flag.enabled
+
+class Flags:
+ def __init__(self, request):
+ self.request = request
+
+ def notifications(self):
+ return (
+ self.request.db.query(AdminFlag)
+ .filter(AdminFlag.enabled.is_(True), AdminFlag.notify.is_(True))
+ .all()
+ )
+
+ def enabled(self, flag_name):
+ flag = self.request.db.query(AdminFlag).get(flag_name)
+ return flag.enabled if flag else False
+
+
+def includeme(config):
+ config.add_request_method(Flags, name='flags', reify=True)
diff --git a/warehouse/admin/routes.py b/warehouse/admin/routes.py
--- a/warehouse/admin/routes.py
+++ b/warehouse/admin/routes.py
@@ -119,3 +119,15 @@ def includeme(config):
"/admin/blacklist/remove/",
domain=warehouse,
)
+
+ # Flags
+ config.add_route(
+ "admin.flags",
+ "/admin/flags/",
+ domain=warehouse,
+ )
+ config.add_route(
+ "admin.flags.edit",
+ "/admin/flags/edit/",
+ domain=warehouse,
+ )
diff --git a/warehouse/admin/views/flags.py b/warehouse/admin/views/flags.py
new file mode 100644
--- /dev/null
+++ b/warehouse/admin/views/flags.py
@@ -0,0 +1,49 @@
+# Licensed under the Apache License, Version 2.0 (the 'License');
+# you may not use this file except in compliance with the License.
+# You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing, software
+# distributed under the License is distributed on an 'AS IS' BASIS,
+# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+# See the License for the specific language governing permissions and
+# limitations under the License.
+
+from pyramid.httpexceptions import HTTPSeeOther
+from pyramid.view import view_config
+
+from warehouse.admin.flags import AdminFlag
+
+
+@view_config(
+ route_name='admin.flags',
+ renderer='admin/flags/index.html',
+ permission='admin',
+ uses_session=True,
+)
+def get_flags(request):
+ return {
+ 'flags': request.db.query(AdminFlag).order_by(AdminFlag.id).all(),
+ }
+
+
+@view_config(
+ route_name='admin.flags.edit',
+ permission='admin',
+ request_method='POST',
+ uses_session=True,
+ require_methods=False,
+ require_csrf=True,
+)
+def edit_flag(request):
+ flag = request.db.query(AdminFlag).get(request.POST['id'])
+ flag.description = request.POST['description']
+ flag.enabled = bool(request.POST.get('enabled'))
+
+ request.session.flash(
+ f'Successfully edited flag {flag.id!r}',
+ queue='success',
+ )
+
+ return HTTPSeeOther(request.route_path('admin.flags'))
diff --git a/warehouse/db.py b/warehouse/db.py
--- a/warehouse/db.py
+++ b/warehouse/db.py
@@ -162,6 +162,12 @@ def cleanup(request):
session.close()
connection.close()
+ # Check if we're in read-only mode
+ from warehouse.admin.flags import AdminFlag
+ flag = session.query(AdminFlag).get('read-only')
+ if flag and flag.enabled and not request.user.is_superuser:
+ request.tm.doom()
+
# Return our session now that it's created and registered
return session
diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -45,7 +45,6 @@
Project, Release, Dependency, DependencyKind, Role, File, Filename,
JournalEntry, BlacklistedProject,
)
-from warehouse.utils.admin_flags import AdminFlag
from warehouse.utils import http
@@ -696,6 +695,13 @@ def _is_duplicate_file(db_session, filename, hashes):
require_methods=["POST"],
)
def file_upload(request):
+ # If we're in read-only mode, let upload clients know
+ if request.flags.enabled('read-only'):
+ raise _exc_with_message(
+ HTTPForbidden,
+ 'Read Only Mode: Uploads are temporarily disabled',
+ )
+
# Before we do anything, if there isn't an authenticated user with this
# request, then we'll go ahead and bomb out.
if request.authenticated_userid is None:
@@ -789,9 +795,7 @@ def file_upload(request):
# Check for AdminFlag set by a PyPI Administrator disabling new project
# registration, reasons for this include Spammers, security
# vulnerabilities, or just wanting to be lazy and not worry ;)
- if AdminFlag.is_enabled(
- request.db,
- 'disallow-new-project-registration'):
+ if request.flags.enabled('disallow-new-project-registration'):
raise _exc_with_message(
HTTPForbidden,
("New Project Registration Temporarily Disabled "
diff --git a/warehouse/migrations/versions/6418f7d86a4b_add_read_only_adminflag.py b/warehouse/migrations/versions/6418f7d86a4b_add_read_only_adminflag.py
new file mode 100644
--- /dev/null
+++ b/warehouse/migrations/versions/6418f7d86a4b_add_read_only_adminflag.py
@@ -0,0 +1,40 @@
+# Licensed under the Apache License, Version 2.0 (the "License");
+# you may not use this file except in compliance with the License.
+# You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing, software
+# distributed under the License is distributed on an "AS IS" BASIS,
+# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+# See the License for the specific language governing permissions and
+# limitations under the License.
+"""
+Add read-only AdminFlag
+
+Revision ID: 6418f7d86a4b
+Revises: 5dda74213989
+Create Date: 2018-03-23 20:51:31.558587
+"""
+
+from alembic import op
+
+
+revision = '6418f7d86a4b'
+down_revision = 'bf73e785eed9'
+
+
+def upgrade():
+ op.execute("""
+ INSERT INTO warehouse_admin_flag(id, description, enabled, notify)
+ VALUES (
+ 'read-only',
+ 'Read Only Mode: Any write operations will have no effect',
+ FALSE,
+ TRUE
+ )
+ """)
+
+
+def downgrade():
+ op.execute("DELETE FROM warehouse_admin_flag WHERE id = 'read-only'")
diff --git a/warehouse/migrations/versions/bf73e785eed9_add_notify_column_to_adminflag.py b/warehouse/migrations/versions/bf73e785eed9_add_notify_column_to_adminflag.py
new file mode 100644
--- /dev/null
+++ b/warehouse/migrations/versions/bf73e785eed9_add_notify_column_to_adminflag.py
@@ -0,0 +1,40 @@
+# Licensed under the Apache License, Version 2.0 (the "License");
+# you may not use this file except in compliance with the License.
+# You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing, software
+# distributed under the License is distributed on an "AS IS" BASIS,
+# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+# See the License for the specific language governing permissions and
+# limitations under the License.
+"""
+Add notify column to AdminFlag
+
+Revision ID: bf73e785eed9
+Revises: 5dda74213989
+Create Date: 2018-03-23 21:20:05.834821
+"""
+
+from alembic import op
+import sqlalchemy as sa
+
+revision = 'bf73e785eed9'
+down_revision = '5dda74213989'
+
+
+def upgrade():
+ op.add_column(
+ 'warehouse_admin_flag',
+ sa.Column(
+ 'notify',
+ sa.Boolean(),
+ server_default=sa.text('false'),
+ nullable=False,
+ ),
+ )
+
+
+def downgrade():
+ op.drop_column('warehouse_admin_flag', 'notify')
| diff --git a/tests/common/db/utils.py b/tests/common/db/admin.py
similarity index 94%
rename from tests/common/db/utils.py
rename to tests/common/db/admin.py
--- a/tests/common/db/utils.py
+++ b/tests/common/db/admin.py
@@ -12,7 +12,7 @@
import factory.fuzzy
-from warehouse.utils.admin_flags import AdminFlag
+from warehouse.admin.flags import AdminFlag
from .base import WarehouseFactory
diff --git a/tests/conftest.py b/tests/conftest.py
--- a/tests/conftest.py
+++ b/tests/conftest.py
@@ -27,6 +27,7 @@
from warehouse.config import configure
from warehouse.accounts import services
+from warehouse.admin.flags import Flags
from .common.db import Session
@@ -215,6 +216,7 @@ def query_recorder(app_config):
def db_request(pyramid_request, db_session, datadog):
pyramid_request.registry.datadog = datadog
pyramid_request.db = db_session
+ pyramid_request.flags = Flags(pyramid_request)
return pyramid_request
diff --git a/tests/unit/accounts/test_views.py b/tests/unit/accounts/test_views.py
--- a/tests/unit/accounts/test_views.py
+++ b/tests/unit/accounts/test_views.py
@@ -25,7 +25,7 @@
IUserService, ITokenService, TokenExpired, TokenInvalid, TokenMissing,
TooManyFailedLogins
)
-from warehouse.utils.admin_flags import AdminFlag
+from warehouse.admin.flags import AdminFlag
from ...common.db.accounts import EmailFactory, UserFactory
@@ -394,11 +394,13 @@ def test_register_redirect(self, db_request, monkeypatch):
assert send_email.calls == [pretend.call(db_request, email)]
def test_register_fails_with_admin_flag_set(self, db_request):
- admin_flag = (db_request.db.query(AdminFlag)
- .filter(
- AdminFlag.id == 'disallow-new-user-registration')
- .first())
- admin_flag.enabled = True
+ # This flag was already set via migration, just need to enable it
+ flag = (
+ db_request.db.query(AdminFlag)
+ .get('disallow-new-user-registration')
+ )
+ flag.enabled = True
+
db_request.method = "POST"
db_request.POST.update({
diff --git a/tests/unit/admin/test_core.py b/tests/unit/admin/test_core.py
--- a/tests/unit/admin/test_core.py
+++ b/tests/unit/admin/test_core.py
@@ -34,7 +34,10 @@ def test_includeme():
assert config.add_static_view.calls == [
pretend.call("admin/static", "static", cache_max_age=0),
]
- assert config.include.calls == [pretend.call(".routes")]
+ assert config.include.calls == [
+ pretend.call(".routes"),
+ pretend.call(".flags"),
+ ]
assert config.add_view.calls == [
pretend.call(
accounts_views.login,
diff --git a/tests/unit/utils/test_admin_flags.py b/tests/unit/admin/test_flags.py
similarity index 57%
rename from tests/unit/utils/test_admin_flags.py
rename to tests/unit/admin/test_flags.py
--- a/tests/unit/utils/test_admin_flags.py
+++ b/tests/unit/admin/test_flags.py
@@ -10,16 +10,15 @@
# See the License for the specific language governing permissions and
# limitations under the License.
-from warehouse.utils.admin_flags import AdminFlag
-
-from ...common.db.utils import AdminFlagFactory as DBAdminFlagFactory
+from ...common.db.admin import AdminFlagFactory
class TestAdminFlag:
- def test_default(self, db_session):
- assert not AdminFlag.is_enabled(db_session, 'not-a-real-flag')
+ def test_default(self, db_request):
+ assert not db_request.flags.enabled('not-a-real-flag')
+
+ def test_enabled(self, db_request):
+ AdminFlagFactory(id='this-flag-is-enabled')
- def test_enabled(self, db_session):
- DBAdminFlagFactory.create(id='this-flag-is-enabled', enabled=True)
- assert AdminFlag.is_enabled(db_session, 'this-flag-is-enabled')
+ assert db_request.flags.enabled('this-flag-is-enabled')
diff --git a/tests/unit/admin/test_routes.py b/tests/unit/admin/test_routes.py
--- a/tests/unit/admin/test_routes.py
+++ b/tests/unit/admin/test_routes.py
@@ -116,4 +116,14 @@ def test_includeme():
"/admin/blacklist/remove/",
domain=warehouse,
),
+ pretend.call(
+ "admin.flags",
+ "/admin/flags/",
+ domain=warehouse,
+ ),
+ pretend.call(
+ "admin.flags.edit",
+ "/admin/flags/edit/",
+ domain=warehouse,
+ ),
]
diff --git a/tests/unit/admin/views/test_flags.py b/tests/unit/admin/views/test_flags.py
new file mode 100644
--- /dev/null
+++ b/tests/unit/admin/views/test_flags.py
@@ -0,0 +1,100 @@
+# Licensed under the Apache License, Version 2.0 (the "License");
+# you may not use this file except in compliance with the License.
+# You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing, software
+# distributed under the License is distributed on an "AS IS" BASIS,
+# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+# See the License for the specific language governing permissions and
+# limitations under the License.
+
+import pytest
+
+from warehouse.admin.flags import AdminFlag
+from warehouse.admin.views import flags as views
+
+from ....common.db.admin import AdminFlagFactory
+
+
+class TestGetFlags:
+
+ def test_get_classifiers(self, db_request):
+ # Clear out any existing flags added from migrations
+ db_request.db.query(AdminFlag).delete()
+
+ flag_a = AdminFlagFactory(id='flag-a')
+ flag_b = AdminFlagFactory(id='flag-b')
+
+ assert views.get_flags(db_request) == {
+ 'flags': [flag_a, flag_b],
+ }
+
+
+class TestEditFlag:
+
+ @pytest.mark.parametrize(
+ "description, enabled, post, expected_description, expected_enabled",
+ [
+ (
+ # Nothing changed when enabled
+ 'old', True,
+ {'id': 'foo-bar', 'description': 'old', 'enabled': 'on'},
+ 'old', True,
+ ),
+ (
+ # Nothing changed when disabled
+ 'old', False,
+ {'id': 'foo-bar', 'description': 'old'},
+ 'old', False,
+ ),
+ (
+ # Enable flag
+ 'old', False,
+ {'id': 'foo-bar', 'description': 'old', 'enabled': 'on'},
+ 'old', True,
+ ),
+ (
+ # Disable flag
+ 'old', True,
+ {'id': 'foo-bar', 'description': 'old'},
+ 'old', False,
+ ),
+ (
+ # Change description when enabled
+ 'old', True,
+ {'id': 'foo-bar', 'description': 'new', 'enabled': 'on'},
+ 'new', True,
+ ),
+ (
+ # Change description when disabled
+ 'old', False,
+ {'id': 'foo-bar', 'description': 'new'},
+ 'new', False,
+ ),
+ ]
+ )
+ def test_edit_flag(
+ self, db_request, description, enabled, post, expected_description,
+ expected_enabled):
+
+ # Clear out any existing flags added from migrations
+ db_request.db.query(AdminFlag).delete()
+
+ flag = AdminFlagFactory(
+ id='foo-bar',
+ description=description,
+ enabled=enabled,
+ )
+
+ db_request.POST = post
+ db_request.route_path = lambda *a: '/the/redirect'
+ db_request.flash = lambda *a: None
+
+ views.edit_flag(db_request)
+
+ db_request.db.flush()
+
+ assert flag.enabled == expected_enabled
+ assert flag.description == expected_description
diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -36,7 +36,7 @@
File, Filename, Dependency, DependencyKind, Release, Project, Role,
JournalEntry,
)
-from warehouse.utils.admin_flags import AdminFlag
+from warehouse.admin.flags import AdminFlag
from ...common.db.accounts import UserFactory, EmailFactory
from ...common.db.packaging import (
@@ -738,6 +738,7 @@ def test_fails_invalid_version(self, pyramid_config, pyramid_request,
version):
pyramid_config.testing_securitypolicy(userid=1)
pyramid_request.POST["protocol_version"] = version
+ pyramid_request.flags = pretend.stub(enabled=lambda *a: False)
with pytest.raises(HTTPBadRequest) as excinfo:
legacy.file_upload(pyramid_request)
@@ -2797,7 +2798,21 @@ def test_upload_purges_legacy(self, pyramid_config, db_request,
),
]
+ def test_fails_in_read_only_mode(self, pyramid_request):
+ pyramid_request.flags = pretend.stub(enabled=lambda *a: True)
+
+ with pytest.raises(HTTPForbidden) as excinfo:
+ legacy.file_upload(pyramid_request)
+
+ resp = excinfo.value
+
+ assert resp.status_code == 403
+ assert resp.status == (
+ '403 Read Only Mode: Uploads are temporarily disabled'
+ )
+
def test_fails_without_user(self, pyramid_config, pyramid_request):
+ pyramid_request.flags = pretend.stub(enabled=lambda *a: False)
pyramid_config.testing_securitypolicy(userid=None)
with pytest.raises(HTTPForbidden) as excinfo:
diff --git a/tests/unit/test_db.py b/tests/unit/test_db.py
--- a/tests/unit/test_db.py
+++ b/tests/unit/test_db.py
@@ -147,7 +147,10 @@ def test_creates_engine(monkeypatch):
],
)
def test_create_session(monkeypatch, read_only, tx_status):
- session_obj = pretend.stub(close=pretend.call_recorder(lambda: None))
+ session_obj = pretend.stub(
+ close=pretend.call_recorder(lambda: None),
+ query=lambda *a: pretend.stub(get=lambda *a: None),
+ )
session_cls = pretend.call_recorder(lambda bind: session_obj)
monkeypatch.setattr(db, "Session", session_cls)
@@ -200,6 +203,57 @@ def test_create_session(monkeypatch, read_only, tx_status):
connection.connection.rollback.calls == [pretend.call()]
[email protected](
+ "admin_flag, is_superuser, doom_calls",
+ [
+ (None, True, []),
+ (None, False, []),
+ (pretend.stub(enabled=False), True, []),
+ (pretend.stub(enabled=False), False, []),
+ (pretend.stub(enabled=True, description='flag description'), True, []),
+ (
+ pretend.stub(enabled=True, description='flag description'),
+ False,
+ [pretend.call()],
+ ),
+ ],
+)
+def test_create_session_read_only_mode(
+ admin_flag, is_superuser, doom_calls, monkeypatch):
+ get = pretend.call_recorder(lambda *a: admin_flag)
+ session_obj = pretend.stub(
+ close=lambda: None,
+ query=lambda *a: pretend.stub(get=get),
+ )
+ session_cls = pretend.call_recorder(lambda bind: session_obj)
+ monkeypatch.setattr(db, "Session", session_cls)
+
+ register = pretend.call_recorder(lambda session, transaction_manager: None)
+ monkeypatch.setattr(zope.sqlalchemy, "register", register)
+
+ connection = pretend.stub(
+ connection=pretend.stub(
+ get_transaction_status=lambda: pretend.stub(),
+ set_session=lambda **kw: None,
+ rollback=lambda: None,
+ ),
+ info={},
+ close=lambda: None,
+ )
+ engine = pretend.stub(connect=pretend.call_recorder(lambda: connection))
+ request = pretend.stub(
+ registry={"sqlalchemy.engine": engine},
+ tm=pretend.stub(doom=pretend.call_recorder(lambda: None)),
+ read_only=False,
+ add_finished_callback=lambda callback: None,
+ user=pretend.stub(is_superuser=is_superuser),
+ )
+
+ assert _create_session(request) is session_obj
+ assert get.calls == [pretend.call('read-only')]
+ assert request.tm.doom.calls == doom_calls
+
+
@pytest.mark.parametrize(
("predicates", "expected"),
[
| Implement a Read Only Flag to prevent any write operations
There are times when we need to prevent any state modification on pypi.python.org but we do not need to prevent reading from the database or other storage areas. It would be great if we could prevent all write operations using a toggle somehow.
| A big red banner across the top of the web UI noting "Maintenance Mode" with a description of what that means would be a nice addition too.
@dstufft Any ideas about where would be the best place to short-circuit this? Perhaps a `@db.listens_for(db.Session, "before_commit")` or something to abort the commit?
``request.tm.doom()`` should allow the request to continue, but abort it at the last minute. | 2018-03-23T21:33:58Z | [] | [] |
pypi/warehouse | 3,396 | pypi__warehouse-3396 | [
"3383"
] | a6d34187a91d4a7f872079d04d26c19eacbcec48 | diff --git a/warehouse/packaging/__init__.py b/warehouse/packaging/__init__.py
--- a/warehouse/packaging/__init__.py
+++ b/warehouse/packaging/__init__.py
@@ -17,7 +17,7 @@
from warehouse.accounts.models import User, Email
from warehouse.cache.origin import key_factory, receive_set
from warehouse.packaging.interfaces import IFileStorage
-from warehouse.packaging.models import Project, Release
+from warehouse.packaging.models import Project, Release, Role
from warehouse.packaging.tasks import compute_trending
@@ -60,6 +60,13 @@ def includeme(config):
key_factory("all-projects"),
],
)
+ config.register_origin_cache_keys(
+ Role,
+ purge_keys=[
+ key_factory("user/{obj.user.username}"),
+ key_factory("project/{obj.project.normalized_name}")
+ ],
+ )
config.register_origin_cache_keys(
User,
cache_keys=["user/{obj.username}"],
| diff --git a/tests/unit/packaging/test_init.py b/tests/unit/packaging/test_init.py
--- a/tests/unit/packaging/test_init.py
+++ b/tests/unit/packaging/test_init.py
@@ -18,7 +18,7 @@
from warehouse import packaging
from warehouse.accounts.models import Email, User
from warehouse.packaging.interfaces import IFileStorage
-from warehouse.packaging.models import Project, Release
+from warehouse.packaging.models import Project, Release, Role
from warehouse.packaging.tasks import compute_trending
@@ -71,6 +71,13 @@ def key_factory(keystring, iterate_on=None):
key_factory("all-projects"),
],
),
+ pretend.call(
+ Role,
+ purge_keys=[
+ key_factory("user/{obj.user.username}"),
+ key_factory("project/{obj.project.normalized_name}")
+ ],
+ ),
pretend.call(
User,
cache_keys=["user/{obj.username}"],
| Missing Purges
Noticed this while setting up new mirror. We don't seem to be purging `project/<normalized_name>` key when projects are deleted.
This leads bandersnatch to get confused and fall behind until the key is purged so the JSON api returns a 404
| Also appears to miss purges due to maintainership changes and _pypi_hidden (legacy) changes.
Legacy doesn't purge Warehouse at all
I think we should just disable editing anything but pypi_hidden on legacy, and disable the journal entry for it then @dstufft ?
fine with me, pypi_hidden is useless in warehouse anyways.
👍
pypi-legacy interference resolved in https://github.com/pypa/pypi-legacy/pull/785
Still need maintainer changes to purge `project/` keys though.
Should just require adding:
```
config.register_origin_cache_keys(
Role,
purge_keys=[
key_factory("user/{obj.user.username}"),
key_factory("project/{obj.project.normalized_name}")
],
)
``` | 2018-03-23T22:40:40Z | [] | [] |
pypi/warehouse | 3,405 | pypi__warehouse-3405 | [
"3185"
] | 56788c28a85b58c4c309db2cc3b802a8622c580b | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -810,7 +810,10 @@ def file_upload(request):
raise _exc_with_message(
HTTPForbidden,
("New Project Registration Temporarily Disabled "
- "See https://pypi.org/help#admin-intervention for details"),
+ "See {projecthelp} for details")
+ .format(
+ projecthelp=request.route_url(
+ 'help', _anchor='admin-intervention')),
) from None
# Ensure that user has at least one verified email address. This should
| diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -1045,6 +1045,10 @@ def test_fails_with_admin_flag_set(self, pyramid_config, db_request):
),
})
+ db_request.route_url = pretend.call_recorder(
+ lambda route, **kw: "/the/help/url/"
+ )
+
with pytest.raises(HTTPForbidden) as excinfo:
legacy.file_upload(db_request)
@@ -1053,7 +1057,7 @@ def test_fails_with_admin_flag_set(self, pyramid_config, db_request):
assert resp.status_code == 403
assert resp.status == ("403 New Project Registration Temporarily "
"Disabled See "
- "https://pypi.org/help#admin-intervention for "
+ "/the/help/url/ for "
"details")
def test_upload_fails_without_file(self, pyramid_config, db_request):
| Make error message (for when the admin intervention flag is set) work with Test PyPI
Right now, when someone tries to upload a package to PyPI but the admin intervention flag is set (meaning that we're under a spam attack and the PyPI admins have temporarily shut off all uploads), the error message we send them includes -- hardcoded -- the URL https://pypi.org. Instead, we should use Pyramid's URL routing to refer to the URL of the PyPI in question, thus ensuring that we send error messages that, as appropriate, refer to pypi.org, test.pypi.org, or any other future PyPI.
To fix this, look at `warehouse/forklift/legacy.py` and `tests/unit/forklift/test_legacy.py` (the test is `test_fails_with_admin_flag_set`), and make changes similar to the changes I made in #3183. If you're used to string substitution/string formatting in Python, this should be a pretty approachable first Warehouse issue.
---
**Good First Issue**: This issue is good for first time contributors. If you've already contributed to Warehouse, please work on [another issue without this label](https://github.com/pypa/warehouse/issues?utf8=%E2%9C%93&q=is%3Aissue+is%3Aopen+-label%3A%22good+first+issue%22) instead. If there is not a corresponding pull request for this issue, it is up for grabs. For directions for getting set up, see our [Getting Started Guide](https://warehouse.pypa.io/development/getting-started/). If you are working on this issue and have questions, please feel free to ask them here, [`#pypa-dev` on Freenode](https://webchat.freenode.net/?channels=%23pypa-dev), or the [pypa-dev mailing list](https://groups.google.com/forum/#!forum/pypa-dev).
| 2018-03-25T02:44:22Z | [] | [] |
|
pypi/warehouse | 3,409 | pypi__warehouse-3409 | [
"2825"
] | 3735086fac4e18955205f9164bd3857456efec8f | diff --git a/warehouse/legacy/api/json.py b/warehouse/legacy/api/json.py
--- a/warehouse/legacy/api/json.py
+++ b/warehouse/legacy/api/json.py
@@ -20,6 +20,26 @@
from warehouse.packaging.models import File, Release
+# Generate appropriate CORS headers for the JSON endpoint.
+# We want to allow Cross-Origin requests here so that users can interact
+# with these endpoints via XHR/Fetch APIs in the browser.
+_CORS_HEADERS = {
+ "Access-Control-Allow-Origin": "*",
+ "Access-Control-Allow-Headers": ", ".join([
+ "Content-Type",
+ "If-Match",
+ "If-Modified-Since",
+ "If-None-Match",
+ "If-Unmodified-Since",
+ ]),
+ "Access-Control-Allow-Methods": "GET",
+ "Access-Control-Max-Age": "86400", # 1 day.
+ "Access-Control-Expose-Headers": ", ".join([
+ "X-PyPI-Last-Serial",
+ ]),
+}
+
+
@view_config(
route_name="legacy.api.json.project",
renderer="json",
@@ -36,6 +56,7 @@ def json_project(project, request):
if project.name != request.matchdict.get("name", project.name):
return HTTPMovedPermanently(
request.current_route_path(name=project.name),
+ headers=_CORS_HEADERS
)
try:
@@ -49,7 +70,7 @@ def json_project(project, request):
.one()
)
except NoResultFound:
- return HTTPNotFound()
+ return HTTPNotFound(headers=_CORS_HEADERS)
return json_release(release, request)
@@ -72,22 +93,11 @@ def json_release(release, request):
if project.name != request.matchdict.get("name", project.name):
return HTTPMovedPermanently(
request.current_route_path(name=project.name),
+ headers=_CORS_HEADERS
)
- # We want to allow CORS here to enable anyone to fetch data from this API
- request.response.headers["Access-Control-Allow-Origin"] = "*"
- request.response.headers["Access-Control-Allow-Headers"] = ", ".join([
- "Content-Type",
- "If-Match",
- "If-Modified-Since",
- "If-None-Match",
- "If-Unmodified-Since",
- ])
- request.response.headers["Access-Control-Allow-Methods"] = "GET"
- request.response.headers["Access-Control-Max-Age"] = "86400"
- request.response.headers["Access-Control-Expose-Headers"] = ", ".join([
- "X-PyPI-Last-Serial",
- ])
+ # Apply CORS headers.
+ request.response.headers.update(_CORS_HEADERS)
# Get the latest serial number for this project.
request.response.headers["X-PyPI-Last-Serial"] = str(project.last_serial)
| diff --git a/tests/unit/legacy/api/test_json.py b/tests/unit/legacy/api/test_json.py
--- a/tests/unit/legacy/api/test_json.py
+++ b/tests/unit/legacy/api/test_json.py
@@ -22,6 +22,17 @@
)
+def _assert_has_cors_headers(headers):
+ assert headers["Access-Control-Allow-Origin"] == "*"
+ assert headers["Access-Control-Allow-Headers"] == (
+ "Content-Type, If-Match, If-Modified-Since, If-None-Match, "
+ "If-Unmodified-Since"
+ )
+ assert headers["Access-Control-Allow-Methods"] == "GET"
+ assert headers["Access-Control-Max-Age"] == "86400"
+ assert headers["Access-Control-Expose-Headers"] == "X-PyPI-Last-Serial"
+
+
class TestJSONProject:
def test_normalizing_redirects(self, db_request):
@@ -40,6 +51,7 @@ def test_normalizing_redirects(self, db_request):
assert isinstance(resp, HTTPMovedPermanently)
assert resp.headers["Location"] == "/project/the-redirect/"
+ _assert_has_cors_headers(resp.headers)
assert db_request.current_route_path.calls == [
pretend.call(name=project.name),
]
@@ -48,6 +60,7 @@ def test_missing_release(self, db_request):
project = ProjectFactory.create()
resp = json.json_project(project, db_request)
assert isinstance(resp, HTTPNotFound)
+ _assert_has_cors_headers(resp.headers)
def test_calls_release_detail(self, monkeypatch, db_request):
project = ProjectFactory.create()
@@ -121,6 +134,7 @@ def test_normalizing_redirects(self, db_request):
assert isinstance(resp, HTTPMovedPermanently)
assert resp.headers["Location"] == "/project/the-redirect/3.0/"
+ _assert_has_cors_headers(resp.headers)
assert db_request.current_route_path.calls == [
pretend.call(name=release.project.name),
]
@@ -165,16 +179,8 @@ def test_detail_renders(self, pyramid_config, db_request):
pretend.call("legacy.docs", project=project.name),
}
- headers = db_request.response.headers
- assert headers["Access-Control-Allow-Origin"] == "*"
- assert headers["Access-Control-Allow-Headers"] == (
- "Content-Type, If-Match, If-Modified-Since, If-None-Match, "
- "If-Unmodified-Since"
- )
- assert headers["Access-Control-Allow-Methods"] == "GET"
- assert headers["Access-Control-Max-Age"] == "86400"
- assert headers["Access-Control-Expose-Headers"] == "X-PyPI-Last-Serial"
- assert headers["X-PyPI-Last-Serial"] == str(je.id)
+ _assert_has_cors_headers(db_request.response.headers)
+ assert db_request.response.headers["X-PyPI-Last-Serial"] == str(je.id)
assert result == {
"info": {
| Missing Access-Control-Allow-Origin in redirect headers
From https://sourceforge.net/p/pypi/support-requests/741/:
> Hi,
>
> Both pypi.org and pypi.python.org do not have proper Access-Control-Allow-Origin response headers in their redirect responses. Please see https://stackoverflow.com/questions/44637138/case-sensitive-url-triggering-cors-error.
>
> It would be very helpful to add these to the headers so that redirect requests are propertly handled.
>
> Thanks!
---
**Good First Issue**: This issue is good for first time contributors. If there is not a corresponding pull request for this issue, it is up for grabs. For directions for getting set up, see our [Getting Started Guide](https://warehouse.pypa.io/development/getting-started/). If you are working on this issue and have questions, please feel free to ask them here, [`#pypa-dev` on Freenode](https://webchat.freenode.net/?channels=%23pypa-dev), or the [pypa-dev mailing list](https://groups.google.com/forum/#!forum/pypa-dev).
| Per [discussion in today's bug triage meeting](https://wiki.python.org/psf/PackagingWG/2018-01-29-Warehouse), this doesn't seem critical, and we think it should be considered a "task" in the canonical URL handling code. | 2018-03-25T04:13:36Z | [] | [] |
pypi/warehouse | 3,413 | pypi__warehouse-3413 | [
"582"
] | 48d4e686974ca929ad85d755b20d6c73d47ea7c0 | diff --git a/warehouse/config.py b/warehouse/config.py
--- a/warehouse/config.py
+++ b/warehouse/config.py
@@ -192,6 +192,7 @@ def configure(settings=None):
default=21600, # 6 hours
)
maybe_set_compound(settings, "files", "backend", "FILES_BACKEND")
+ maybe_set_compound(settings, "docs", "backend", "DOCS_BACKEND")
maybe_set_compound(settings, "origin_cache", "backend", "ORIGIN_CACHE")
# Add the settings we use when the environment is set to development.
diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -1216,7 +1216,7 @@ def file_upload(request):
# this won't take affect until after a commit has happened, for
# now we'll just ignore it and save it before the transaction is
# committed.
- storage = request.find_service(IFileStorage)
+ storage = request.find_service(IFileStorage, name='files')
storage.store(
file_.path,
os.path.join(tmpdir, filename),
diff --git a/warehouse/manage/views.py b/warehouse/manage/views.py
--- a/warehouse/manage/views.py
+++ b/warehouse/manage/views.py
@@ -31,7 +31,11 @@
SaveAccountForm,
)
from warehouse.packaging.models import File, JournalEntry, Project, Role
-from warehouse.utils.project import confirm_project, remove_project
+from warehouse.utils.project import (
+ confirm_project,
+ destroy_docs,
+ remove_project,
+)
@view_defaults(
@@ -358,6 +362,26 @@ def delete_project(project, request):
return HTTPSeeOther(request.route_path('manage.projects'))
+@view_config(
+ route_name="manage.project.destroy_docs",
+ uses_session=True,
+ require_methods=["POST"],
+ permission="manage",
+)
+def destroy_project_docs(project, request):
+ confirm_project(
+ project, request, fail_route="manage.project.documentation"
+ )
+ destroy_docs(project, request)
+
+ return HTTPSeeOther(
+ request.route_path(
+ 'manage.project.documentation',
+ project_name=project.normalized_name,
+ )
+ )
+
+
@view_config(
route_name="manage.project.releases",
renderer="manage/releases.html",
@@ -757,3 +781,15 @@ def manage_project_history(project, request):
'project': project,
'journals': journals,
}
+
+
+@view_config(
+ route_name="manage.project.documentation",
+ renderer="manage/documentation.html",
+ uses_session=True,
+ permission="manage",
+)
+def manage_project_documentation(project, request):
+ return {
+ 'project': project,
+ }
diff --git a/warehouse/packaging/__init__.py b/warehouse/packaging/__init__.py
--- a/warehouse/packaging/__init__.py
+++ b/warehouse/packaging/__init__.py
@@ -36,10 +36,19 @@ def email_primary_receive_set(config, target, value, oldvalue, initiator):
def includeme(config):
# Register whatever file storage backend has been configured for storing
# our package files.
- storage_class = config.maybe_dotted(
+ files_storage_class = config.maybe_dotted(
config.registry.settings["files.backend"],
)
- config.register_service_factory(storage_class.create_service, IFileStorage)
+ config.register_service_factory(
+ files_storage_class.create_service, IFileStorage, name='files'
+ )
+
+ docs_storage_class = config.maybe_dotted(
+ config.registry.settings["docs.backend"],
+ )
+ config.register_service_factory(
+ docs_storage_class.create_service, IFileStorage, name='docs'
+ )
# Register our origin cache keys
config.register_origin_cache_keys(
diff --git a/warehouse/packaging/interfaces.py b/warehouse/packaging/interfaces.py
--- a/warehouse/packaging/interfaces.py
+++ b/warehouse/packaging/interfaces.py
@@ -33,3 +33,8 @@ def store(path, file_path, *, meta=None):
specified by path. An additional meta keyword argument may contain
extra information that an implementation may or may not store.
"""
+
+ def remove_by_prefix(prefix):
+ """
+ Remove all files matching the given prefix.
+ """
diff --git a/warehouse/packaging/services.py b/warehouse/packaging/services.py
--- a/warehouse/packaging/services.py
+++ b/warehouse/packaging/services.py
@@ -11,6 +11,7 @@
# limitations under the License.
import os.path
+import shutil
import warnings
import botocore.exceptions
@@ -51,6 +52,13 @@ def store(self, path, file_path, *, meta=None):
with open(file_path, "rb") as src_fp:
dest_fp.write(src_fp.read())
+ def remove_by_prefix(self, prefix):
+ directory = os.path.join(self.base, prefix)
+ try:
+ shutil.rmtree(directory)
+ except FileNotFoundError:
+ pass
+
@implementer(IFileStorage)
class S3FileStorage:
@@ -97,3 +105,15 @@ def store(self, path, file_path, *, meta=None):
path = self._get_path(path)
self.bucket.upload_file(file_path, path, ExtraArgs=extra_args)
+
+ def remove_by_prefix(self, prefix):
+ if self.prefix:
+ prefix = os.path.join(self.prefix, prefix)
+ keys_to_delete = []
+ for key in self.bucket.list(prefix=prefix):
+ keys_to_delete.append(key)
+ if len(keys_to_delete) > 99:
+ self.bucket.delete_keys(keys_to_delete)
+ keys_to_delete = []
+ if len(keys_to_delete) > 0:
+ self.bucket.delete_keys(keys_to_delete)
diff --git a/warehouse/routes.py b/warehouse/routes.py
--- a/warehouse/routes.py
+++ b/warehouse/routes.py
@@ -134,6 +134,13 @@ def includeme(config):
traverse="/{project_name}",
domain=warehouse,
)
+ config.add_route(
+ "manage.project.destroy_docs",
+ "/manage/project/{project_name}/delete_project_docs/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{project_name}",
+ domain=warehouse,
+ )
config.add_route(
"manage.project.releases",
"/manage/project/{project_name}/releases/",
@@ -169,6 +176,13 @@ def includeme(config):
traverse="/{project_name}",
domain=warehouse,
)
+ config.add_route(
+ "manage.project.documentation",
+ "/manage/project/{project_name}/documentation/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{project_name}",
+ domain=warehouse,
+ )
config.add_route(
"manage.project.history",
"/manage/project/{project_name}/history/",
diff --git a/warehouse/utils/project.py b/warehouse/utils/project.py
--- a/warehouse/utils/project.py
+++ b/warehouse/utils/project.py
@@ -13,9 +13,21 @@
from packaging.utils import canonicalize_name
from pyramid.httpexceptions import HTTPSeeOther
+from warehouse.tasks import task
+from warehouse.packaging.interfaces import IFileStorage
from warehouse.packaging.models import JournalEntry
+@task(bind=True, ignore_result=True, acks_late=True)
+def remove_documentation(task, request, project_name):
+ request.log.info("Removing documentation for %s", project_name)
+ storage = request.find_service(IFileStorage, name='docs')
+ try:
+ storage.remove_by_prefix(project_name)
+ except Exception as exc:
+ task.retry(exc=exc)
+
+
def confirm_project(project, request, fail_route):
confirm = request.POST.get("confirm_project_name")
project_name = project.normalized_name
@@ -61,3 +73,24 @@ def remove_project(project, request, flash=True):
f"Successfully deleted the project {project.name!r}.",
queue="success",
)
+
+
+def destroy_docs(project, request, flash=True):
+ request.db.add(
+ JournalEntry(
+ name=project.name,
+ action="docdestroy",
+ submitted_by=request.user,
+ submitted_from=request.remote_addr,
+ )
+ )
+
+ request.task(remove_documentation).delay(project.name)
+
+ project.has_docs = False
+
+ if flash:
+ request.session.flash(
+ f"Successfully deleted docs for project {project.name!r}.",
+ queue="success",
+ )
| diff --git a/tests/conftest.py b/tests/conftest.py
--- a/tests/conftest.py
+++ b/tests/conftest.py
@@ -117,6 +117,7 @@ def app_config(database):
"ratelimit.url": "memory://",
"elasticsearch.url": "https://localhost/warehouse",
"files.backend": "warehouse.packaging.services.LocalFileStorage",
+ "docs.backend": "warehouse.packaging.services.LocalFileStorage",
"files.url": "http://localhost:7000/",
"sessions.secret": "123456",
"sessions.url": "redis://localhost:0/",
diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -1196,13 +1196,15 @@ def storage_service_store(path, file_path, *, meta):
storage_service = pretend.stub(store=storage_service_store)
db_request.find_service = pretend.call_recorder(
- lambda svc: storage_service
+ lambda svc, name=None: storage_service
)
resp = legacy.file_upload(db_request)
assert resp.status_code == 200
- assert db_request.find_service.calls == [pretend.call(IFileStorage)]
+ assert db_request.find_service.calls == [
+ pretend.call(IFileStorage, name="files"),
+ ]
assert len(storage_service.store.calls) == 2 if has_signature else 1
assert storage_service.store.calls[0] == pretend.call(
"/".join([
@@ -2110,7 +2112,7 @@ def storage_service_store(path, file_path, *, meta):
storage_service = pretend.stub(store=storage_service_store)
db_request.find_service = pretend.call_recorder(
- lambda svc: storage_service
+ lambda svc, name=None: storage_service
)
monkeypatch.setattr(
@@ -2121,7 +2123,9 @@ def storage_service_store(path, file_path, *, meta):
resp = legacy.file_upload(db_request)
assert resp.status_code == 200
- assert db_request.find_service.calls == [pretend.call(IFileStorage)]
+ assert db_request.find_service.calls == [
+ pretend.call(IFileStorage, name="files"),
+ ]
assert storage_service.store.calls == [
pretend.call(
"/".join([
@@ -2218,7 +2222,7 @@ def storage_service_store(path, file_path, *, meta):
storage_service = pretend.stub(store=storage_service_store)
db_request.find_service = pretend.call_recorder(
- lambda svc: storage_service
+ lambda svc, name=None: storage_service
)
monkeypatch.setattr(
@@ -2229,7 +2233,9 @@ def storage_service_store(path, file_path, *, meta):
resp = legacy.file_upload(db_request)
assert resp.status_code == 200
- assert db_request.find_service.calls == [pretend.call(IFileStorage)]
+ assert db_request.find_service.calls == [
+ pretend.call(IFileStorage, name="files"),
+ ]
assert storage_service.store.calls == [
pretend.call(
"/".join([
@@ -2315,7 +2321,7 @@ def storage_service_store(path, file_path, *, meta):
assert fp.read() == b"A fake file."
storage_service = pretend.stub(store=storage_service_store)
- db_request.find_service = lambda svc: storage_service
+ db_request.find_service = lambda svc, name=None: storage_service
monkeypatch.setattr(
legacy,
@@ -2361,7 +2367,7 @@ def storage_service_store(path, file_path, *, meta):
assert fp.read() == b"A fake file."
storage_service = pretend.stub(store=storage_service_store)
- db_request.find_service = lambda svc: storage_service
+ db_request.find_service = lambda svc, name=None: storage_service
monkeypatch.setattr(
legacy,
@@ -2463,7 +2469,7 @@ def test_upload_succeeds_creates_release(self, pyramid_config, db_request):
])
storage_service = pretend.stub(store=lambda path, filepath, meta: None)
- db_request.find_service = lambda svc: storage_service
+ db_request.find_service = lambda svc, name=None: storage_service
resp = legacy.file_upload(db_request)
@@ -2554,7 +2560,7 @@ def test_equivalent_version_one_release(self, pyramid_config, db_request):
})
storage_service = pretend.stub(store=lambda path, filepath, meta: None)
- db_request.find_service = lambda svc: storage_service
+ db_request.find_service = lambda svc, name=None: storage_service
resp = legacy.file_upload(db_request)
@@ -2601,7 +2607,7 @@ def test_equivalent_canonical_versions(self, pyramid_config, db_request):
})
storage_service = pretend.stub(store=lambda path, filepath, meta: None)
- db_request.find_service = lambda svc: storage_service
+ db_request.find_service = lambda svc, name=None: storage_service
legacy.file_upload(db_request)
@@ -2631,7 +2637,7 @@ def test_upload_succeeds_creates_project(self, pyramid_config, db_request):
})
storage_service = pretend.stub(store=lambda path, filepath, meta: None)
- db_request.find_service = lambda svc: storage_service
+ db_request.find_service = lambda svc, name=None: storage_service
db_request.remote_addr = "10.10.10.10"
resp = legacy.file_upload(db_request)
@@ -2733,7 +2739,7 @@ def test_upload_requires_verified_email(self, pyramid_config, db_request,
})
storage_service = pretend.stub(store=lambda path, filepath, meta: None)
- db_request.find_service = lambda svc: storage_service
+ db_request.find_service = lambda svc, name=None: storage_service
db_request.remote_addr = "10.10.10.10"
if expected_success:
@@ -2777,7 +2783,7 @@ def test_upload_purges_legacy(self, pyramid_config, db_request,
})
storage_service = pretend.stub(store=lambda path, filepath, meta: None)
- db_request.find_service = lambda svc: storage_service
+ db_request.find_service = lambda svc, name=None: storage_service
db_request.remote_addr = "10.10.10.10"
tm = pretend.stub(
@@ -2873,7 +2879,7 @@ def test_autohides_old_releases(self, pyramid_config, db_request):
])
storage_service = pretend.stub(store=lambda path, filepath, meta: None)
- db_request.find_service = lambda svc: storage_service
+ db_request.find_service = lambda svc, name=None: storage_service
resp = legacy.file_upload(db_request)
@@ -2954,7 +2960,7 @@ def test_doesnt_autohides_old_releases(self, pyramid_config, db_request):
])
storage_service = pretend.stub(store=lambda path, filepath, meta: None)
- db_request.find_service = lambda svc: storage_service
+ db_request.find_service = lambda svc, name=None: storage_service
resp = legacy.file_upload(db_request)
diff --git a/tests/unit/manage/test_views.py b/tests/unit/manage/test_views.py
--- a/tests/unit/manage/test_views.py
+++ b/tests/unit/manage/test_views.py
@@ -23,6 +23,7 @@
from warehouse.manage import views
from warehouse.accounts.interfaces import IUserService
from warehouse.packaging.models import JournalEntry, Project, Role, User
+from warehouse.utils.project import remove_documentation
from ...common.db.accounts import EmailFactory
from ...common.db.packaging import (
@@ -830,6 +831,105 @@ def test_delete_project(self, db_request):
.filter(Project.name == "foo").count())
+class TestManageProjectDocumentation:
+
+ def test_manage_project_documentation(self):
+ request = pretend.stub()
+ project = pretend.stub()
+
+ assert views.manage_project_documentation(project, request) == {
+ "project": project,
+ }
+
+ def test_destroy_project_docs_no_confirm(self):
+ project = pretend.stub(normalized_name='foo')
+ request = pretend.stub(
+ POST={},
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ ),
+ route_path=lambda *a, **kw: "/foo/bar/",
+ )
+
+ with pytest.raises(HTTPSeeOther) as exc:
+ views.destroy_project_docs(project, request)
+ assert exc.value.status_code == 303
+ assert exc.value.headers["Location"] == "/foo/bar/"
+
+ assert request.session.flash.calls == [
+ pretend.call("Must confirm the request.", queue="error"),
+ ]
+
+ def test_destroy_project_docs_wrong_confirm(self):
+ project = pretend.stub(normalized_name='foo')
+ request = pretend.stub(
+ POST={"confirm_project_name": "bar"},
+ session=pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ ),
+ route_path=lambda *a, **kw: "/foo/bar/",
+ )
+
+ with pytest.raises(HTTPSeeOther) as exc:
+ views.destroy_project_docs(project, request)
+ assert exc.value.status_code == 303
+ assert exc.value.headers["Location"] == "/foo/bar/"
+
+ assert request.session.flash.calls == [
+ pretend.call(
+ "Could not delete project - 'bar' is not the same as 'foo'",
+ queue="error"
+ ),
+ ]
+
+ def test_destroy_project_docs(self, db_request):
+ project = ProjectFactory.create(name="foo")
+ remove_documentation_recorder = pretend.stub(
+ delay=pretend.call_recorder(
+ lambda *a, **kw: None
+ )
+ )
+ task = pretend.call_recorder(
+ lambda *a, **kw: remove_documentation_recorder
+ )
+
+ db_request.route_path = pretend.call_recorder(
+ lambda *a, **kw: "/the-redirect"
+ )
+ db_request.session = pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None),
+ )
+ db_request.POST["confirm_project_name"] = project.normalized_name
+ db_request.user = UserFactory.create()
+ db_request.remote_addr = "192.168.1.1"
+ db_request.task = task
+
+ result = views.destroy_project_docs(project, db_request)
+
+ assert task.calls == [
+ pretend.call(remove_documentation)
+ ]
+
+ assert remove_documentation_recorder.delay.calls == [
+ pretend.call(project.name)
+ ]
+
+ assert db_request.session.flash.calls == [
+ pretend.call(
+ "Successfully deleted docs for project 'foo'.",
+ queue="success"
+ ),
+ ]
+ assert db_request.route_path.calls == [
+ pretend.call('manage.project.documentation', project_name='foo'),
+ ]
+ assert isinstance(result, HTTPSeeOther)
+ assert result.headers["Location"] == "/the-redirect"
+ assert not (db_request.db.query(Project)
+ .filter(Project.name == "foo")
+ .first().has_docs)
+
+
class TestManageProjectReleases:
def test_manage_project_releases(self):
diff --git a/tests/unit/packaging/test_init.py b/tests/unit/packaging/test_init.py
--- a/tests/unit/packaging/test_init.py
+++ b/tests/unit/packaging/test_init.py
@@ -34,11 +34,12 @@ def key_factory(keystring, iterate_on=None):
config = pretend.stub(
maybe_dotted=lambda dotted: storage_class,
register_service_factory=pretend.call_recorder(
- lambda factory, iface: None,
+ lambda factory, iface, name=None: None,
),
registry=pretend.stub(
settings={
"files.backend": "foo.bar",
+ "docs.backend": "wu.tang",
},
),
register_origin_cache_keys=pretend.call_recorder(lambda c, **kw: None),
@@ -50,7 +51,8 @@ def key_factory(keystring, iterate_on=None):
packaging.includeme(config)
assert config.register_service_factory.calls == [
- pretend.call(storage_class.create_service, IFileStorage),
+ pretend.call(storage_class.create_service, IFileStorage, name='files'),
+ pretend.call(storage_class.create_service, IFileStorage, name='docs'),
]
assert config.register_origin_cache_keys.calls == [
pretend.call(
diff --git a/tests/unit/packaging/test_services.py b/tests/unit/packaging/test_services.py
--- a/tests/unit/packaging/test_services.py
+++ b/tests/unit/packaging/test_services.py
@@ -87,6 +87,52 @@ def test_stores_two_files(self, tmpdir):
with open(os.path.join(storage_dir, "foo/second.txt"), "rb") as fp:
assert fp.read() == b"Second Test File!"
+ def test_delete_by_prefix(self, tmpdir):
+ filename0 = str(tmpdir.join("testfile0.txt"))
+ with open(filename0, "wb") as fp:
+ fp.write(b"Zeroth Test File!")
+
+ filename1 = str(tmpdir.join("testfile1.txt"))
+ with open(filename1, "wb") as fp:
+ fp.write(b"First Test File!")
+
+ filename2 = str(tmpdir.join("testfile2.txt"))
+ with open(filename2, "wb") as fp:
+ fp.write(b"Second Test File!")
+
+ storage_dir = str(tmpdir.join("storage"))
+ storage = LocalFileStorage(storage_dir)
+ storage.store("foo/zeroth.txt", filename0)
+ storage.store("foo/first.txt", filename1)
+ storage.store("bar/second.txt", filename2)
+
+ with open(os.path.join(storage_dir, "foo/zeroth.txt"), "rb") as fp:
+ assert fp.read() == b"Zeroth Test File!"
+
+ with open(os.path.join(storage_dir, "foo/first.txt"), "rb") as fp:
+ assert fp.read() == b"First Test File!"
+
+ with open(os.path.join(storage_dir, "bar/second.txt"), "rb") as fp:
+ assert fp.read() == b"Second Test File!"
+
+ storage.remove_by_prefix('foo')
+
+ with pytest.raises(FileNotFoundError):
+ storage.get("foo/zeroth.txt")
+
+ with pytest.raises(FileNotFoundError):
+ storage.get("foo/first.txt")
+
+ with open(os.path.join(storage_dir, "bar/second.txt"), "rb") as fp:
+ assert fp.read() == b"Second Test File!"
+
+ def test_delete_already_gone(self, tmpdir):
+ storage_dir = str(tmpdir.join("storage"))
+ storage = LocalFileStorage(storage_dir)
+
+ response = storage.remove_by_prefix('foo')
+ assert response is None
+
class TestS3FileStorage:
@@ -229,3 +275,60 @@ def test_hashed_path_without_prefix(self):
assert file_object.read() == b"my contents"
assert bucket.Object.calls == [pretend.call("ab/file.txt")]
+
+ @pytest.mark.parametrize('file_count', [66, 100])
+ def test_delete_by_prefix(self, file_count):
+ files = [f'foo/{i}.html' for i in range(file_count)]
+ bucket = pretend.stub(
+ list=pretend.call_recorder(lambda prefix=None: files),
+ delete_keys=pretend.call_recorder(lambda keys: None),
+ )
+ storage = S3FileStorage(bucket)
+
+ storage.remove_by_prefix('foo')
+
+ assert bucket.list.calls == [
+ pretend.call(prefix='foo'),
+ ]
+
+ assert bucket.delete_keys.calls == [
+ pretend.call([f'foo/{i}.html' for i in range(file_count)]),
+ ]
+
+ def test_delete_by_prefix_more_files(self):
+ files = [f'foo/{i}.html' for i in range(150)]
+ bucket = pretend.stub(
+ list=pretend.call_recorder(lambda prefix=None: files),
+ delete_keys=pretend.call_recorder(lambda keys: None),
+ )
+ storage = S3FileStorage(bucket)
+
+ storage.remove_by_prefix('foo')
+
+ assert bucket.list.calls == [
+ pretend.call(prefix='foo'),
+ ]
+
+ assert bucket.delete_keys.calls == [
+ pretend.call([f'foo/{i}.html' for i in range(100)]),
+ pretend.call([f'foo/{i}.html' for i in range(100, 150)]),
+ ]
+
+ def test_delete_by_prefix_with_storage_prefix(self):
+ files = [f'docs/foo/{i}.html' for i in range(150)]
+ bucket = pretend.stub(
+ list=pretend.call_recorder(lambda prefix=None: files),
+ delete_keys=pretend.call_recorder(lambda keys: None),
+ )
+ storage = S3FileStorage(bucket, prefix='docs')
+
+ storage.remove_by_prefix('foo')
+
+ assert bucket.list.calls == [
+ pretend.call(prefix='docs/foo'),
+ ]
+
+ assert bucket.delete_keys.calls == [
+ pretend.call([f'docs/foo/{i}.html' for i in range(100)]),
+ pretend.call([f'docs/foo/{i}.html' for i in range(100, 150)]),
+ ]
diff --git a/tests/unit/test_routes.py b/tests/unit/test_routes.py
--- a/tests/unit/test_routes.py
+++ b/tests/unit/test_routes.py
@@ -168,6 +168,13 @@ def add_policy(name, filename):
traverse="/{project_name}",
domain=warehouse,
),
+ pretend.call(
+ "manage.project.destroy_docs",
+ "/manage/project/{project_name}/delete_project_docs/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{project_name}",
+ domain=warehouse,
+ ),
pretend.call(
"manage.project.releases",
"/manage/project/{project_name}/releases/",
@@ -203,6 +210,13 @@ def add_policy(name, filename):
traverse="/{project_name}",
domain=warehouse,
),
+ pretend.call(
+ "manage.project.documentation",
+ "/manage/project/{project_name}/documentation/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{project_name}",
+ domain=warehouse,
+ ),
pretend.call(
"manage.project.history",
"/manage/project/{project_name}/history/",
diff --git a/tests/unit/utils/test_project.py b/tests/unit/utils/test_project.py
--- a/tests/unit/utils/test_project.py
+++ b/tests/unit/utils/test_project.py
@@ -11,13 +11,18 @@
# limitations under the License.
import pytest
-from pretend import call, call_recorder, stub
+from pretend import call, call_recorder, stub, raiser
from pyramid.httpexceptions import HTTPSeeOther
from warehouse.packaging.models import (
Project, Release, Dependency, File, Role, JournalEntry
)
-from warehouse.utils.project import confirm_project, remove_project
+from warehouse.utils.project import (
+ confirm_project,
+ destroy_docs,
+ remove_project,
+ remove_documentation,
+)
from ...common.db.accounts import UserFactory
from ...common.db.packaging import (
@@ -134,3 +139,90 @@ def test_remove_project(db_request, flash):
assert journal_entry.action == "remove"
assert journal_entry.submitted_by == db_request.user
assert journal_entry.submitted_from == db_request.remote_addr
+
+
[email protected](
+ 'flash',
+ [True, False]
+)
+def test_destroy_docs(db_request, flash):
+ user = UserFactory.create()
+ project = ProjectFactory.create(name="foo", has_docs=True)
+ RoleFactory.create(user=user, project=project)
+
+ db_request.user = user
+ db_request.remote_addr = "192.168.1.1"
+ db_request.session = stub(flash=call_recorder(lambda *a, **kw: stub()))
+ remove_documentation_recorder = stub(
+ delay=call_recorder(lambda *a, **kw: None)
+ )
+ db_request.task = call_recorder(
+ lambda *a, **kw: remove_documentation_recorder
+ )
+
+ destroy_docs(project, db_request, flash=flash)
+
+ journal_entry = (
+ db_request.db.query(JournalEntry)
+ .filter(JournalEntry.name == "foo")
+ .one()
+ )
+ assert journal_entry.action == "docdestroy"
+ assert journal_entry.submitted_by == db_request.user
+ assert journal_entry.submitted_from == db_request.remote_addr
+
+ assert not (db_request.db.query(Project)
+ .filter(Project.name == project.name)
+ .first().has_docs)
+
+ assert remove_documentation_recorder.delay.calls == [
+ call('foo')
+ ]
+
+ if flash:
+ assert db_request.session.flash.calls == [
+ call(
+ "Successfully deleted docs for project 'foo'.",
+ queue="success"
+ ),
+ ]
+ else:
+ assert db_request.session.flash.calls == []
+
+
+def test_remove_documentation(db_request):
+ project = ProjectFactory.create(name="foo", has_docs=True)
+ task = stub()
+ service = stub(remove_by_prefix=call_recorder(lambda project_name: None))
+ db_request.find_service = call_recorder(
+ lambda interface, name=None: service
+ )
+ db_request.log = stub(info=call_recorder(lambda *a, **kw: None))
+
+ remove_documentation(task, db_request, project.name)
+
+ assert service.remove_by_prefix.calls == [
+ call(project.name)
+ ]
+
+ assert db_request.log.info.calls == [
+ call("Removing documentation for %s", project.name),
+ ]
+
+
+def test_remove_documentation_retry(db_request):
+ project = ProjectFactory.create(name="foo", has_docs=True)
+ task = stub(retry=call_recorder(lambda *a, **kw: None))
+ service = stub(remove_by_prefix=raiser(Exception))
+ db_request.find_service = call_recorder(
+ lambda interface, name=None: service
+ )
+ db_request.log = stub(info=call_recorder(lambda *a, **kw: None))
+
+ remove_documentation(task, db_request, project.name)
+
+ assert len(task.retry.calls) == 1
+
+ assert db_request.log.info.calls == [
+ call("Removing documentation for %s", project.name),
+ ]
| Add the ability to delete "legacy" documentation
If a project has existing legacy documentation hosted on pythonhosted.org, we should allow them to delete this documentation. This should come with appropriate warnings that this is a one time, destructive operation that cannot be undone, that new uploads of documentation are no longer allowed and that they should not do it until they've moved their documentation hosting elsewhere.
We should probably include at least a suggestion of using ReadTheDocs here.
This should also provide people with the option to add a redirect when deleting their documentation so that they can redirect to their new location.
| @dstufft - is this required for shut down, or can we move it to post launch?
It can be post shut down, it doesn't currently exist.
Actually I lied-- We're not allowing new docs to be uploaded in Warehouse, so we'll want people to be able to delete their existing documentation so they can migrate to something else before we shut down legacy.
Posting very late to an old thread, but adding my support for adding this ability. For `more-itertools` we added docs to RTD, but the old ones still show up on Google and there's a link to them on PyPI.
Oh, there's a button for this! Never mind.
>> This should also provide people with the option to add a redirect when deleting their documentation so that they can redirect to their new location.
Is there any redirect option yet? I've erased my pythonhosted docs (which can't be updated) and moved my docs elsewhere, but there are published academic papers and other materials that refer to documentation for my package as being on pythonhosted.org. Currently, following any of those links just returns an error page with no guidance of where to go next. I'm sure many users can eventually figure out where to go with Google, but it would be much better if pythonhosted allowed for a way to redirect or at least list the location of the updated docs.
Let me second @computron's suggestion - it would be really nice to be able to redirect from the pythonhosted.org docs to our new docs now hosted on readthedocs.org. Thanks much.
Wow. Seriously? This is such a major oversight. You expect me to choose between a bad option:
- Delete the pythonhosted.org page, so that my user base can't find it.
and a worse option:
- Live with two docs pages, one of them out-dated, which for a while will show up with higher search rankings?
Furthermore, google search offers almost no search results regarding this issue. It took me over an hour to find this. At least make this info available on the pypi edit project page. PLEASE.
@dstufft, as per @lingthio's comment above, and the distutils-sig thread at https://mail.python.org/pipermail/distutils-sig/2017-September/031453.html, this is no longer a potential future problem, it's an *actual* problem right now: with pypi.org providing the only artifact upload interface, there's no longer any way for package maintainers to update their pythonhosted.org documentation.
Mere deletion isn't adequate, since that doesn't allow previously published links to be transparently redirected (preferable), or at least to reach a "These docs are no longer here, go to [other page] instead" page.
As prior art for handling this more gracefully, https://docs.readthedocs.io/en/latest/user-defined-redirects.html would be worth looking at.
It may even be worth chatting to @ericholscher to see whether or not it might be possible to migrate the pythonhosted.org pages into ReadTheDocs as static HTML, such that the redirect strategy literally *is* to use RTD's existing support for this.
As a compromise between adding a new complex interaction for user-defined redirects and/or parsing special links out of descriptions/metadata, would it be sufficient simply to redirect pythonhosted docs for any package missing them to the corresponding package page? Then at least if the project has a Documentation or Home Page link in their description or metadata the user can continue by following it to the current docs.
Redirecting to an URL in the metadata would be nicer, either Home-page or a specific Project-URL (and the latter would give projects more control on where the documentation link redirects to).
In the longer run #1419, which is mostly about being able to add links to the sidebar on PyPI.org through Project-URL, is a nicer solution.
P.S. For a long time I didn't have links to documentation in my long-description because PyPI legacy automatically ads a link to documentation hosted on pythonhosted.org. I only recently added links to the long description because that doesn't work with warehouse and I couldn't get the same functionality through Project-URL links.
I'd be interested in helping get something set up.
I've added a space for this in the new UI:
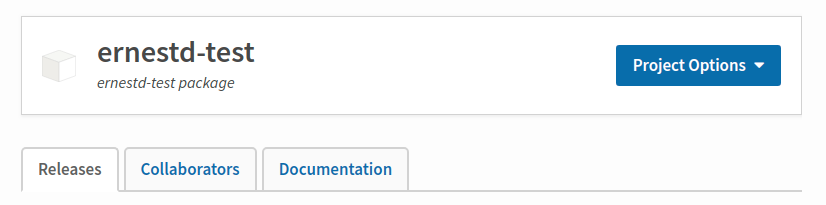
For now, this tab will be commented out, but this interface should give us the flexibility to add a specific documentation page and address this issue.
FYI, Project-URLs have been fixed in #2813.
This is In Progress. Implementing the Delete Docs button is one part, but there is a larger story.
A discussion happened late last year with Read The Docs (RTD) about what a good approach for handling a link between documentation and the package index might be, as warehouse isn't well suited to serve as a documentation host moving forward.
The summary is that post launch, we may be able to implement some sort of handoff to "introduce" a user to RTD for the purposes of authenticating them as valid owners of a namespace on their site that indicates "canonical" package documentation hosting.
Implementing a "redirect" functionality is still up in the air, as it would effectively communicate that we're not going to do _anything_ for package doc hosting moving forward... which hasn't been decided. Although I agree that it would be a good escape hatch in the interim before a more full fledged solution is implemented.
Once we have this issue resolved, we should ping `@jezdez` and `@WoLpH` to update the [sphinx-pypi-upload](https://bitbucket.org/jezdez/sphinx-pypi-upload/) project & [fork](https://github.com/WoLpH/sphinx-pypi-upload/) ([relevant PR](https://github.com/WoLpH/sphinx-pypi-upload/pull/2)) appropriately.
For context, since I think a few people subscribed to this issue don't know:
Warehouse, the codebase behind the new PyPI, is available as a pre-production site at https://pypi.org . Thanks to [limited funding from Mozilla's Open Source Support Program](https://pyfound.blogspot.com/2017/11/the-psf-awarded-moss-grant-pypi.html), and it's on its way to replacing the legacy PyPI site by the end of April ([roadmap](https://wiki.python.org/psf/WarehouseRoadmap)). [We're currently seeking feedback from package maintainers](https://wiki.python.org/psf/WarehousePackageMaintainerTesting), about what does or doesn't work for you in the new interface, and in the next few weeks we'll be doing so with the wider Python developer community.
While the pythonhosted docs/Read the Docs "handoff" can’t really happen until we shut down legacy PyPI, we will be implementing the "delete existing docs" functionality once we (mostly Ernest) have completed the current foundational infrastructure work necessary for the Warehouse switchover. So you'll probably see this within the next six weeks.
I'm sorry for the delay.
@dstufft said,
> We're not allowing new docs to be uploaded in Warehouse, so we'll want people to be able to delete their existing documentation so they can migrate to something else before we shut down legacy.
Does shutting down pypi.python.org also involve shutting down https://www.pythonhosted.org/ and thus making pythonhosted.org documentation (example: https://pythonhosted.org/spyder/help.html ) completely unavailable to users? I presume it does not, in which case, this feature, though deeply needed, does not need to block the shutdown of legacy PyPI.
| 2018-03-25T18:07:47Z | [] | [] |
pypi/warehouse | 3,457 | pypi__warehouse-3457 | [
"3455"
] | cb5764b5d83d81b31543cee275e4bc511d7f8fe8 | diff --git a/warehouse/packaging/tasks.py b/warehouse/packaging/tasks.py
--- a/warehouse/packaging/tasks.py
+++ b/warehouse/packaging/tasks.py
@@ -18,7 +18,7 @@
@tasks.task(ignore_result=True, acks_late=True)
def compute_trending(request):
bq = request.find_service(name="gcloud.bigquery")
- query = bq.run_sync_query(
+ query = bq.query(
""" SELECT project,
IF(
STDDEV(downloads) > 0,
@@ -56,21 +56,11 @@ def compute_trending(request):
ORDER BY zscore DESC
""".format(table=request.registry.settings["warehouse.trending_table"])
)
- query.use_legacy_sql = False
- query.run()
zscores = {}
- page_token = None
- while True:
- rows, total_rows, page_token = query.fetch_data(
- max_results=1000,
- page_token=page_token,
- )
-
- zscores.update(dict(rows))
-
- if not page_token:
- break
+ for row in query.result():
+ row = dict(row)
+ zscores[row["project"]] = row["zscore"]
# We're going to "reset" all of our zscores to a steady state where they
# are all equal to ``None``. The next query will then set any that have a
| diff --git a/tests/unit/packaging/test_tasks.py b/tests/unit/packaging/test_tasks.py
--- a/tests/unit/packaging/test_tasks.py
+++ b/tests/unit/packaging/test_tasks.py
@@ -13,6 +13,8 @@
import pretend
import pytest
+from google.cloud.bigquery import Row
+
from warehouse.cache.origin import IOriginCache
from warehouse.packaging.models import Project
from warehouse.packaging.tasks import compute_trending
@@ -30,18 +32,22 @@ def test_computes_trending(self, db_request, with_purges):
]
results = iter([
- ([(projects[1].normalized_name, 2)], 2, "blah"),
- ([(projects[2].normalized_name, -1)], 2, None),
+ Row(
+ (projects[1].normalized_name, 2),
+ {'project': 0, 'zscore': 1},
+ ),
+ Row(
+ (projects[2].normalized_name, -1),
+ {'project': 0, 'zscore': 1},
+ ),
])
query = pretend.stub(
- use_legacy_sql=True,
- run=pretend.call_recorder(lambda: None),
- fetch_data=pretend.call_recorder(
- lambda max_results, page_token: next(results),
+ result=pretend.call_recorder(
+ lambda *a, **kw: results,
)
)
bigquery = pretend.stub(
- run_sync_query=pretend.call_recorder(lambda q: query),
+ query=pretend.call_recorder(lambda q: query),
)
cacher = pretend.stub(purge=pretend.call_recorder(lambda keys: None))
@@ -62,7 +68,7 @@ def find_service(iface=None, name=None):
compute_trending(db_request)
- assert bigquery.run_sync_query.calls == [
+ assert bigquery.query.calls == [
pretend.call(""" SELECT project,
IF(
STDDEV(downloads) > 0,
@@ -100,8 +106,7 @@ def find_service(iface=None, name=None):
ORDER BY zscore DESC
"""),
]
- assert not query.use_legacy_sql
- assert query.run.calls == [pretend.call()]
+ assert query.result.calls == [pretend.call()]
assert (cacher.purge.calls ==
([pretend.call(["trending"])] if with_purges else []))
| Trending Projects are not updated
I think in the past month that I've been looking at pypi.org (and even before that), the "Trending Projects" haven't changed.
| Looking further, as someone not familiar with the codebase, it seems like the `compute_trending` task isn't setup to run every day or something like that.
Potentially another reason for #3252.
https://github.com/pypa/warehouse/blob/ed8d6a03d86e794b83ccd4e41d0c5b6b7865b8bf/warehouse/packaging/__init__.py#L112-L115
```
pypi-warehouse-worker-beat-856f489568-kjc9b worker-beat [2018-03-28 03:00:00,064: INFO/MainProcess] Loading 1 tasks
pypi-warehouse-worker-beat-856f489568-kjc9b worker-beat [2018-03-28 03:00:00,071: INFO/MainProcess] Scheduler: Sending due task warehouse.packaging.tasks.compute_trending() (warehouse.packaging.tasks.compute_trending)
```
I do see the task being enqueued.
```
pypi-warehouse-worker-7b6dddfb-tlm4s worker FileNotFoundError: [Errno 2] No such file or directory: '/app/gcloud.json'
```
Looks like pathing is goof'd! will fixup!
That was half the issue :)
Second half is they changed the API! https://github.com/GoogleCloudPlatform/google-cloud-python/blob/cd9b89ead0be95827c9eac5a9a8c5d8fdaee3cae/bigquery/CHANGELOG.md#interface-changes--breaking-changes-1
```
pypi-warehouse-worker-5c996d6869-6hbkc worker [2018-03-28 16:03:30,528: ERROR/ForkPoolWorker-2] Task warehouse.packaging.tasks.compute_trending[e6d5d345-bfb9-4fd1-a535-af52c2b1a495] raised unexpected: AttributeError("'Client' object has no attribute 'run_sync_query'",)
pypi-warehouse-worker-5c996d6869-6hbkc worker Traceback (most recent call last):
pypi-warehouse-worker-5c996d6869-6hbkc worker File "/opt/warehouse/lib/python3.6/site-packages/celery/app/trace.py", line 374, in trace_task
pypi-warehouse-worker-5c996d6869-6hbkc worker R = retval = fun(*args, **kwargs)
pypi-warehouse-worker-5c996d6869-6hbkc worker File "/opt/warehouse/src/warehouse/tasks.py", line 70, in __call__
pypi-warehouse-worker-5c996d6869-6hbkc worker return super().__call__(*(self.get_request(),) + args, **kwargs)
pypi-warehouse-worker-5c996d6869-6hbkc worker File "/opt/warehouse/lib/python3.6/site-packages/celery/app/trace.py", line 629, in __protected_call__
pypi-warehouse-worker-5c996d6869-6hbkc worker return self.run(*args, **kwargs)
pypi-warehouse-worker-5c996d6869-6hbkc worker File "/opt/warehouse/src/warehouse/tasks.py", line 58, in run
pypi-warehouse-worker-5c996d6869-6hbkc worker return original_run(*args, **kwargs)
pypi-warehouse-worker-5c996d6869-6hbkc worker File "/opt/warehouse/src/warehouse/packaging/tasks.py", line 21, in compute_trending
pypi-warehouse-worker-5c996d6869-6hbkc worker query = bq.run_sync_query(
pypi-warehouse-worker-5c996d6869-6hbkc worker AttributeError: 'Client' object has no attribute 'run_sync_query'
```
(@pradyunsg -- *good catch* and thanks for the report!) | 2018-03-28T16:57:26Z | [] | [] |
pypi/warehouse | 3,475 | pypi__warehouse-3475 | [
"3474"
] | afee6c3bb957ad222d2a8d6b648c5460c74a7e38 | diff --git a/warehouse/admin/views/journals.py b/warehouse/admin/views/journals.py
--- a/warehouse/admin/views/journals.py
+++ b/warehouse/admin/views/journals.py
@@ -37,7 +37,9 @@ def journals_list(request):
journals_query = (
request.db.query(JournalEntry)
- .order_by(JournalEntry.submitted_date.desc())
+ .order_by(
+ JournalEntry.submitted_date.desc(),
+ JournalEntry.id.desc())
)
if q:
diff --git a/warehouse/admin/views/projects.py b/warehouse/admin/views/projects.py
--- a/warehouse/admin/views/projects.py
+++ b/warehouse/admin/views/projects.py
@@ -105,7 +105,10 @@ def project_detail(project, request):
for entry in (
request.db.query(JournalEntry)
.filter(JournalEntry.name == project.name)
- .order_by(JournalEntry.submitted_date.desc())
+ .order_by(
+ JournalEntry.submitted_date.desc(),
+ JournalEntry.id.desc(),
+ )
.limit(30)
)
]
@@ -208,7 +211,9 @@ def journals_list(project, request):
journals_query = (request.db.query(JournalEntry)
.filter(JournalEntry.name == project.name)
- .order_by(JournalEntry.submitted_date.desc()))
+ .order_by(
+ JournalEntry.submitted_date.desc(),
+ JournalEntry.id.desc()))
if q:
terms = shlex.split(q)
diff --git a/warehouse/legacy/api/xmlrpc/views.py b/warehouse/legacy/api/xmlrpc/views.py
--- a/warehouse/legacy/api/xmlrpc/views.py
+++ b/warehouse/legacy/api/xmlrpc/views.py
@@ -346,7 +346,7 @@ def changelog(request, since, with_ids=False):
entries = (
request.db.query(JournalEntry)
.filter(JournalEntry.submitted_date > since)
- .order_by(JournalEntry.submitted_date)
+ .order_by(JournalEntry.id)
.limit(50000)
)
diff --git a/warehouse/manage/views.py b/warehouse/manage/views.py
--- a/warehouse/manage/views.py
+++ b/warehouse/manage/views.py
@@ -774,7 +774,7 @@ def manage_project_history(project, request):
journals = (
request.db.query(JournalEntry)
.filter(JournalEntry.name == project.name)
- .order_by(JournalEntry.submitted_date.desc())
+ .order_by(JournalEntry.submitted_date.desc(), JournalEntry.id.desc())
.all()
)
return {
diff --git a/warehouse/packaging/models.py b/warehouse/packaging/models.py
--- a/warehouse/packaging/models.py
+++ b/warehouse/packaging/models.py
@@ -362,7 +362,7 @@ def __table_args__(cls): # noqa
secondaryjoin=lambda: (
(User.username == orm.foreign(JournalEntry._submitted_by))
),
- order_by=lambda: JournalEntry.submitted_date.desc(),
+ order_by=lambda: JournalEntry.id.desc(),
# TODO: We have uselist=False here which raises a warning because
# multiple items were returned. This should only be temporary because
# we should add a nullable FK to JournalEntry so we don't need to rely
| diff --git a/tests/unit/admin/views/test_journals.py b/tests/unit/admin/views/test_journals.py
--- a/tests/unit/admin/views/test_journals.py
+++ b/tests/unit/admin/views/test_journals.py
@@ -29,7 +29,7 @@ class TestProjectList:
def test_no_query(self, db_request):
journals = sorted(
[JournalEntryFactory.create() for _ in range(30)],
- key=lambda j: j.submitted_date,
+ key=lambda j: (j.submitted_date, j.id),
reverse=True,
)
result = views.journals_list(db_request)
@@ -42,7 +42,7 @@ def test_no_query(self, db_request):
def test_with_page(self, db_request):
journals = sorted(
[JournalEntryFactory.create() for _ in range(30)],
- key=lambda j: j.submitted_date,
+ key=lambda j: (j.submitted_date, j.id),
reverse=True,
)
db_request.GET["page"] = "2"
@@ -65,7 +65,7 @@ def test_query_basic(self, db_request):
journals0 = sorted(
[JournalEntryFactory.create(name=project0.normalized_name)
for _ in range(30)],
- key=lambda j: j.submitted_date,
+ key=lambda j: (j.submitted_date, j.id),
reverse=True,
)
[JournalEntryFactory.create(name=project1.normalized_name)
@@ -85,7 +85,7 @@ def test_query_term_project(self, db_request):
journals0 = sorted(
[JournalEntryFactory.create(name=project0.normalized_name)
for _ in range(30)],
- key=lambda j: j.submitted_date,
+ key=lambda j: (j.submitted_date, j.id),
reverse=True,
)
[JournalEntryFactory.create(name=project1.normalized_name)
@@ -105,7 +105,7 @@ def test_query_term_user(self, db_request):
journals0 = sorted(
[JournalEntryFactory.create(submitted_by=user0)
for _ in range(30)],
- key=lambda j: j.submitted_date,
+ key=lambda j: (j.submitted_date, j.id),
reverse=True,
)
[JournalEntryFactory.create(submitted_by=user1)
@@ -139,13 +139,13 @@ def test_query_term_ip(self, db_request):
journals0 = sorted(
[JournalEntryFactory.create(submitted_from=ipv4)
for _ in range(10)],
- key=lambda j: j.submitted_date,
+ key=lambda j: (j.submitted_date, j.id),
reverse=True,
)
journals1 = sorted(
[JournalEntryFactory.create(submitted_from=ipv6)
for _ in range(10)],
- key=lambda j: j.submitted_date,
+ key=lambda j: (j.submitted_date, j.id),
reverse=True,
)
diff --git a/tests/unit/admin/views/test_projects.py b/tests/unit/admin/views/test_projects.py
--- a/tests/unit/admin/views/test_projects.py
+++ b/tests/unit/admin/views/test_projects.py
@@ -96,7 +96,7 @@ def test_gets_project(self, db_request):
journals = sorted(
[JournalEntryFactory(name=project.name)
for _ in range(75)],
- key=lambda x: x.submitted_date,
+ key=lambda x: (x.submitted_date, x.id),
reverse=True,
)
roles = sorted(
@@ -257,7 +257,7 @@ def test_no_query(self, db_request):
journals = sorted(
[JournalEntryFactory(name=project.name)
for _ in range(30)],
- key=lambda x: x.submitted_date,
+ key=lambda x: (x.submitted_date, x.id),
reverse=True,
)
db_request.matchdict["project_name"] = project.normalized_name
@@ -274,7 +274,7 @@ def test_with_page(self, db_request):
journals = sorted(
[JournalEntryFactory(name=project.name)
for _ in range(30)],
- key=lambda x: x.submitted_date,
+ key=lambda x: (x.submitted_date, x.id),
reverse=True,
)
db_request.matchdict["project_name"] = project.normalized_name
@@ -300,7 +300,7 @@ def test_version_query(self, db_request):
journals = sorted(
[JournalEntryFactory(name=project.name)
for _ in range(30)],
- key=lambda x: x.submitted_date,
+ key=lambda x: (x.submitted_date, x.id),
reverse=True,
)
db_request.matchdict["project_name"] = project.normalized_name
@@ -318,7 +318,7 @@ def test_invalid_key_query(self, db_request):
journals = sorted(
[JournalEntryFactory(name=project.name)
for _ in range(30)],
- key=lambda x: x.submitted_date,
+ key=lambda x: (x.submitted_date, x.id),
reverse=True,
)
db_request.matchdict["project_name"] = project.normalized_name
@@ -336,7 +336,7 @@ def test_basic_query(self, db_request):
journals = sorted(
[JournalEntryFactory(name=project.name)
for _ in range(30)],
- key=lambda x: x.submitted_date,
+ key=lambda x: (x.submitted_date, x.id),
reverse=True,
)
db_request.matchdict["project_name"] = project.normalized_name
diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -1255,7 +1255,7 @@ def storage_service_store(path, file_path, *, meta):
# Ensure that all of our journal entries have been created
journals = (
db_request.db.query(JournalEntry)
- .order_by("submitted_date")
+ .order_by("submitted_date", "id")
.all()
)
assert [
@@ -2160,7 +2160,7 @@ def storage_service_store(path, file_path, *, meta):
# Ensure that all of our journal entries have been created
journals = (
db_request.db.query(JournalEntry)
- .order_by("submitted_date")
+ .order_by("submitted_date", "id")
.all()
)
assert [
@@ -2270,7 +2270,7 @@ def storage_service_store(path, file_path, *, meta):
# Ensure that all of our journal entries have been created
journals = (
db_request.db.query(JournalEntry)
- .order_by("submitted_date")
+ .order_by("submitted_date", "id")
.all()
)
assert [
@@ -2506,7 +2506,7 @@ def test_upload_succeeds_creates_release(self, pyramid_config, db_request):
# Ensure that all of our journal entries have been created
journals = (
db_request.db.query(JournalEntry)
- .order_by("submitted_date")
+ .order_by("submitted_date", "id")
.all()
)
assert [
@@ -2679,7 +2679,7 @@ def test_upload_succeeds_creates_project(self, pyramid_config, db_request):
# Ensure that all of our journal entries have been created
journals = (
db_request.db.query(JournalEntry)
- .order_by("submitted_date")
+ .order_by("submitted_date", "id")
.all()
)
assert [
diff --git a/tests/unit/legacy/api/xmlrpc/test_xmlrpc.py b/tests/unit/legacy/api/xmlrpc/test_xmlrpc.py
--- a/tests/unit/legacy/api/xmlrpc/test_xmlrpc.py
+++ b/tests/unit/legacy/api/xmlrpc/test_xmlrpc.py
@@ -525,7 +525,7 @@ def test_changelog(db_request, with_ids):
for _ in range(10):
entries.append(JournalEntryFactory.create(name=project.name))
- entries = sorted(entries, key=lambda x: x.submitted_date)
+ entries = sorted(entries, key=lambda x: x.id)
since = int(
entries[int(len(entries) / 2)].submitted_date
| Simultaneous events are not ordered consistently on project history page
Sometimes the "new release" event ends up after (above) the first upload of that release, other times it's the other way around:
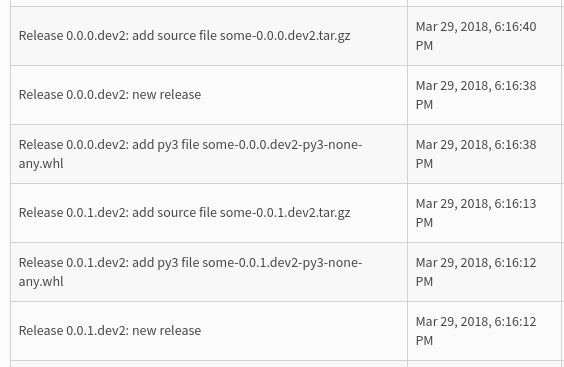
(as reported in #pypa-dev)
| 2018-03-30T16:30:20Z | [] | [] |
|
pypi/warehouse | 3,498 | pypi__warehouse-3498 | [
"3430"
] | 7b95f860e21da1feab160d825ce6053a69c83fc4 | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -760,19 +760,22 @@ def file_upload(request):
field_name = sorted(form.errors.keys())[0]
if field_name in form:
- if form[field_name].description:
+ field = form[field_name]
+ if field.description and isinstance(field, wtforms.StringField):
error_message = (
"{value!r} is an invalid value for {field}. ".format(
- value=form[field_name].data,
- field=form[field_name].description) +
+ value=field.data,
+ field=field.description) +
"Error: {} ".format(form.errors[field_name][0]) +
"see "
"https://packaging.python.org/specifications/core-metadata"
)
else:
- error_message = "{field}: {msgs[0]}".format(
- field=field_name,
- msgs=form.errors[field_name],
+ error_message = (
+ "Invalid value for {field}. Error: {msgs[0]}".format(
+ field=field_name,
+ msgs=form.errors[field_name],
+ )
)
else:
error_message = "Error: {}".format(form.errors[field_name][0])
| diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -814,7 +814,7 @@ def test_fails_invalid_version(self, pyramid_config, pyramid_request,
"version": "1.0",
"md5_digest": "bad",
},
- "filetype: This field is required.",
+ "Invalid value for filetype. Error: This field is required.",
),
(
{
@@ -835,7 +835,7 @@ def test_fails_invalid_version(self, pyramid_config, pyramid_request,
"pyversion": "1.0",
"md5_digest": "bad",
},
- "filetype: Unknown type of file.",
+ "Invalid value for filetype. Error: Unknown type of file.",
),
(
{
@@ -867,8 +867,8 @@ def test_fails_invalid_version(self, pyramid_config, pyramid_request,
"filetype": "sdist",
"sha256_digest": "an invalid sha256 digest",
},
- "sha256_digest: "
- "Must be a valid, hex encoded, SHA256 message digest."
+ "Invalid value for sha256_digest. "
+ "Error: Must be a valid, hex encoded, SHA256 message digest."
),
# summary errors
@@ -1494,11 +1494,9 @@ def test_upload_fails_with_invalid_classifier(self, pyramid_config,
assert resp.status_code == 400
assert resp.status == (
- "400 ['Environment :: Other Environment'] "
- "is an invalid value for Classifier. "
+ "400 Invalid value for classifiers. "
"Error: 'Environment :: Other Environment' is not a valid choice "
- "for this field "
- "see https://packaging.python.org/specifications/core-metadata"
+ "for this field"
)
@pytest.mark.parametrize(
| Improved error message when attempting upload to Warehouse via twine with an invalid trove classifier
I just now uploaded a new release to PyPI. Initially, I had made a typo in one of my trove classifiers (`Topic :: Testing` instead of `Topic :: Software Development :: Testing`).
The error returned by `twine` when I attempted to upload to Warehouse was mostly unhelpful, other than indicating something was wrong with my classifiers:
```
HTTPError: 400 Client Error: ['License :: OSI Approved :: MIT License', 'Natural Language :: English', 'Intended Audience :: Developers', 'Operating System :: OS Independent', 'Programming Language :: Python :: 3 :: Only', 'Programming Language :: Python :: 3.4', 'Programming Languag for url: https://upload.pypi.org/legacy/
```
The error returned by `twine` when I attempted an upload to `testpypi`, though, pointed me (eventually) to the problematic classifier:
```
HTTPError: 400 Client Error: ['License :: OSI Approved :: MIT License', 'Natural Language :: English', 'Intended Audience :: Developers', 'Operating System :: OS Independent', 'Programming Language :: Python :: 3 :: Only', 'Programming Language :: Python :: 3.4', 'Programming Language :: Python :: 3.5', 'Programming Language :: Python :: 3.6', 'Topic :: Software Development :: Libraries :: Python Modules', 'Topic :: Testing', 'Development Status :: 4 - Beta'] is an invalid value for Classifier. Error: 'Topic :: Testing' is not a valid choice for this field see https://packaging.python.org/specifications/core-metadata for url: https://test.pypi.org/legacy/
```
A more succinct, targeted error message here would be hugely helpful.
FWIW, it almost looks like Warehouse tried to generate the same message as legacy, but it got clipped?
| https://github.com/pypa/warehouse/blob/e7bacffd8bb505b3811750f3b4e366ab1b3d157d/warehouse/forklift/legacy.py#L415-L417 is the field we'd need to update. I _think_ we could improve the error message with a custom validator?
Thanks, @bskinn. I just want to make sure -- are you saying this is a regression and worse behavior, compared to legacy PyPI?
@brainwane Yes, it appears to be a regression -- with legacy PyPI, the full error message was echoed to console, and so I was at least able to immediately see the offending classifier, even if I had to stare at it for a bit to find the relevant part, at the end. | 2018-04-02T00:34:49Z | [] | [] |
pypi/warehouse | 3,522 | pypi__warehouse-3522 | [
"3482"
] | 23633d57c1d4b349c6b27907e598fb0d755d6fe3 | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -1097,11 +1097,16 @@ def file_upload(request):
return Response()
elif is_duplicate is not None:
raise _exc_with_message(
- HTTPBadRequest, "The filename or contents already exist. "
- "See " +
- request.route_url(
- 'help', _anchor='file-name-reuse'
- )
+ HTTPBadRequest,
+ # Note: Changing this error message to something that doesn't
+ # start with "File already exists" will break the
+ # --skip-existing functionality in twine
+ # ref: https://github.com/pypa/warehouse/issues/3482
+ # ref: https://github.com/pypa/twine/issues/332
+ "File already exists. See " +
+ request.route_url(
+ 'help', _anchor='file-name-reuse'
+ )
)
# Check to see if the file that was uploaded exists in our filename log
| diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -1835,10 +1835,7 @@ def test_upload_fails_with_existing_filename_diff_content(self,
pretend.call('help', _anchor='file-name-reuse')
]
assert resp.status_code == 400
- assert resp.status == (
- "400 The filename or contents already exist. "
- "See /the/help/url/"
- )
+ assert resp.status == "400 File already exists. See /the/help/url/"
def test_upload_fails_with_diff_filename_same_blake2(self,
pyramid_config,
@@ -1897,10 +1894,7 @@ def test_upload_fails_with_diff_filename_same_blake2(self,
pretend.call('help', _anchor='file-name-reuse')
]
assert resp.status_code == 400
- assert resp.status == (
- "400 The filename or contents already exist. "
- "See /the/help/url/"
- )
+ assert resp.status == "400 File already exists. See /the/help/url/"
def test_upload_fails_with_wrong_filename(self, pyramid_config,
db_request):
| #3310 makes twine --skip-existing option useless
`twine` checks for message starting with `File already exists`:
https://github.com/pypa/twine/blob/bcb73e025102565c395e0bf322faebd7f72e76f7/twine/commands/upload.py#L58-L61
Message has been changed to one starting with `The filename or contents already exist` in #3310:
https://github.com/pypa/warehouse/blob/969695d030aef8b11d5b052e8731a80746c404c8/warehouse/forklift/legacy.py#L1083-L1094
c.f. pypa/twine#332
c.f. failing deployment: https://travis-ci.org/mayeut/gcovr/builds/360424325
| @sigmavirus24 @jonparrott @di I'm offline much of today, could one of you take a look at this?
Thanks for the report, @mayeut.
@brainwane, given you're comment in https://github.com/pypa/twine/issues/332#issuecomment-377689258 saying it shall probably be fixed in `twine`, I guess one thing that could be done is add a comment in warehouse. Maybe something like:
`# changing the message might break twine --skip-existing`
This will prevent further issues from popping up. At least, there can be some coordination before an issue arises (e.g. release twine with new message support then change the message).
With my `twine` hat on, I've always been of the opinion that our current checking around this is fragile and sucks. If Warehouse can provide us with something better that would be fantastic. It seems like Warehouse, however, has traded one plain-text string for another. For this API, I understand returning plain-text/html, but I think if the client says they accept JSON, a JSON payload would be leagues better. Just my 2cents
Hmm, this is unfortunate. Seems like we should revert the error message change so that this behavior is restored for older Twine clients, and then work on improving the brittleness of this in Twine. | 2018-04-02T18:14:11Z | [] | [] |
pypi/warehouse | 3,524 | pypi__warehouse-3524 | [
"3473"
] | d2d989c0a3c71c9b84688e9dacae1fdb73c285bd | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -722,7 +722,7 @@ def file_upload(request):
# string "UNKNOWN". This is basically never what anyone actually wants so
# we'll just go ahead and delete anything whose value is UNKNOWN.
for key in list(request.POST):
- if request.POST.get(key) == "UNKNOWN":
+ if str(request.POST.get(key)).strip() == "UNKNOWN":
del request.POST[key]
# We require protocol_version 1, it's the only supported version however
| diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -1074,11 +1074,14 @@ def test_upload_fails_without_file(self, pyramid_config, db_request):
assert resp.status_code == 400
assert resp.status == "400 Upload payload does not have a file."
- def test_upload_cleans_unknown_values(self, pyramid_config, db_request):
+ @pytest.mark.parametrize("value", [('UNKNOWN'), ('UNKNOWN\n\n')])
+ def test_upload_cleans_unknown_values(
+ self, pyramid_config, db_request, value):
+
pyramid_config.testing_securitypolicy(userid=1)
db_request.POST = MultiDict({
"metadata_version": "1.2",
- "name": "UNKNOWN",
+ "name": value,
"version": "1.0",
"filetype": "sdist",
"md5_digest": "a fake md5 digest",
| Missing long_description sometimes results in "UNKNOWN" project description on Warehouse
Some packages get a nice banner when there's no long_description ([example](https://pypi.org/project/ncep-client/1.0.0/)):
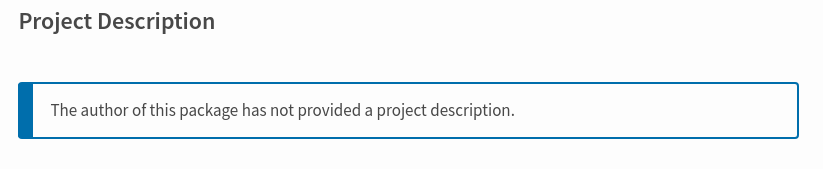
but some just get "UNKNOWN" ([example](https://test.pypi.org/project/some/0.2/) on testPyPI):
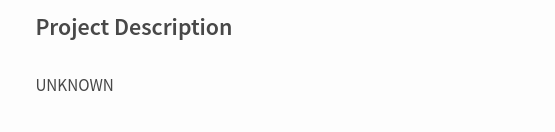
The package with the banner only has an sdist, the one with `UNKNOWN` has both an sdist and a wheel, and the wheel was uploaded first. Both were uploaded within the last couple of days.
| Sounds like a bug in the stripping of ``UNKNOWN`` during upload.
https://github.com/pypa/readme_renderer/pull/69 will help with this once it's merged/released.
This looks like a duplicate of (or related to) #69.
@anowlcalledjosh How did you generate the wheel for [this release](https://test.pypi.org/project/some/0.2/#files)? It's a little weird because it contains a description with extra newlines at the end:
```
$ cat some-0.2.dist-info/DESCRIPTION.rst
UNKNOWN
$ cat some-0.2.dist-info/METADATA
Metadata-Version: 2.0
Name: some
Version: 0.2
Summary: UNKNOWN
Home-page: UNKNOWN
Author: UNKNOWN
Author-email: UNKNOWN
License: UNKNOWN
Platform: UNKNOWN
UNKNOWN
```
which is throwing off this check: https://github.com/pypa/warehouse/blob/090c9c9f59941d819092d3f8ca555dc01400b452/warehouse/forklift/legacy.py#L721-L726
@di That particular release was with an almost empty setup.py (and no setup.cfg). I reproduced it with the following package versions:
- pip 9.0.3
- pkg-resources 0.0.0
- setuptools 39.0.1
- wheel 0.31.0
and a setup.py that looks like this:
```python
from setuptools import setup
setup(
name="some-test",
version="0.0.1",
)
```
and the command `python setup.py bdist_wheel`.
(With wheel 0.31.0, the DESCRIPTION.rst file is not generated any more, but METADATA still ends with a couple of blank lines.)
If I specify a single-line long_description in setup.py, the generated description still ends with two blank lines; however, giving a longer long_description, for example:
```python
long_description="""foo bar
this is a test
it has multiple lines"""
```
results in only a single trailing blank line:
```
$ cat dist/some_test-0.0.1.dist-info/METADATA
Metadata-Version: 2.0
Name: some-test
Version: 0.0.1
Summary: UNKNOWN
Home-page: UNKNOWN
Author: UNKNOWN
Author-email: UNKNOWN
License: UNKNOWN
Platform: UNKNOWN
foo bar
this is a test
it has multiple lines
``` | 2018-04-02T18:28:42Z | [] | [] |
pypi/warehouse | 3,525 | pypi__warehouse-3525 | [
"3428"
] | cc8fb61556d01c5309fa06c335e97d868cc96ab7 | diff --git a/warehouse/utils/wsgi.py b/warehouse/utils/wsgi.py
--- a/warehouse/utils/wsgi.py
+++ b/warehouse/utils/wsgi.py
@@ -48,15 +48,6 @@ def __call__(self, environ, start_response):
)
host = environ.get("HTTP_X_FORWARDED_HOST", "")
- # If we have a X-Forwarded-Port and it disagreed with
- # X-Forwarded-Proto then we're going to listen to X-Forwarded-Port
- # instead. This is because h2o overwrites X-Forwarded-Proto but not
- # X-Forwarded-Port
- # TODO: Note, this can go away if/once h2o/h2o#883 is solved.
- port = environ.get("HTTP_X_FORWARDED_PORT", "")
- if port == "443":
- proto = "https"
-
# Put the new header values into our environment.
if remote_addr:
environ["REMOTE_ADDR"] = remote_addr
| diff --git a/tests/unit/utils/test_wsgi.py b/tests/unit/utils/test_wsgi.py
--- a/tests/unit/utils/test_wsgi.py
+++ b/tests/unit/utils/test_wsgi.py
@@ -149,30 +149,6 @@ def test_selects_right_x_forwarded_value(self):
),
]
- def test_accepts_x_forwarded_port(self):
- response = pretend.stub()
- app = pretend.call_recorder(lambda e, s: response)
-
- environ = {
- "HTTP_X_FORWARDED_PROTO": "http",
- "HTTP_X_FORWARDED_PORT": "443",
- "HTTP_SOME_OTHER_HEADER": "woop",
- }
- start_response = pretend.stub()
-
- resp = wsgi.ProxyFixer(app, token=None)(environ, start_response)
-
- assert resp is response
- assert app.calls == [
- pretend.call(
- {
- "HTTP_SOME_OTHER_HEADER": "woop",
- "wsgi.url_scheme": "https",
- },
- start_response,
- ),
- ]
-
class TestVhmRootRemover:
| Remove X-Forwarded-Proto/X-Forwarded-Port h2o workaround
[In `warehouse/utils/wsgi.py`](https://github.com/pypa/warehouse/blob/master/warehouse/utils/wsgi.py#L51-L58) we note that once https://github.com/h2o/h2o/issues/883 is solved, we can remove a few lines about `X-Forwarded-Port` and `X-Forwarded-Proto`. They resolved that issue in May 2016 and [have released several new versions since then](https://h2o.examp1e.net/). OK to remove workaround?
Followup to b8b9f385382cd659750c694cf8b1b3db6f1f6d35 .
| We don't even use h2o anymore, so it can just be removed I think | 2018-04-02T18:55:16Z | [] | [] |
pypi/warehouse | 3,533 | pypi__warehouse-3533 | [
"3184"
] | f58f87b51ef09688e2393d05b2426a50aafd4a8b | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -823,11 +823,14 @@ def file_upload(request):
if not any(email.verified for email in request.user.emails):
raise _exc_with_message(
HTTPBadRequest,
- ("User {!r} has no verified email addresses, please verify "
- "at least one address before registering a new project on "
- "PyPI. See https://pypi.org/help/#verified-email "
- "for more information.")
- .format(request.user.username),
+ ("User {!r} has no verified email addresses, "
+ "please verify at least one address before registering "
+ "a new project on PyPI. See {projecthelp} "
+ "for more information.").format(
+ request.user.username,
+ projecthelp=request.route_url(
+ 'help', _anchor='verified-email'
+ )),
) from None
# Before we create the project, we're going to check our blacklist to
| diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -2746,16 +2746,24 @@ def test_upload_requires_verified_email(self, pyramid_config, db_request,
resp = legacy.file_upload(db_request)
assert resp.status_code == 200
else:
+ db_request.route_url = pretend.call_recorder(
+ lambda route, **kw: "/the/help/url/"
+ )
+
with pytest.raises(HTTPBadRequest) as excinfo:
legacy.file_upload(db_request)
+
resp = excinfo.value
+
+ assert db_request.route_url.calls == [
+ pretend.call('help', _anchor='verified-email')
+ ]
assert resp.status_code == 400
assert resp.status == (
("400 User {!r} has no verified email "
"addresses, please verify at least one "
"address before registering a new project "
- "on PyPI. See "
- "https://pypi.org/help/#verified-email "
+ "on PyPI. See /the/help/url/ "
"for more information.").format(user.username)
)
| Make error message (for missing verified email) work with Test PyPI
Right now, when someone tries to upload a package to PyPI but does not have a verified email address on file, the error message we send them includes -- hardcoded -- the URL https://pypi.org. Instead, we should use Pyramid's URL routing to refer to the URL of the PyPI in question, thus ensuring that we send error messages that, as appropriate, refer to pypi.org, test.pypi.org, or any other future PyPI.
To fix this, look at `warehouse/forklift/legacy.py` and `tests/unit/forklift/test_legacy.py` (the test is `test_upload_requires_verified_email`), and make changes similar to the changes I made in #3183. If you're used to string substitution/string formatting in Python, this should be a pretty approachable first Warehouse issue.
---
**Good First Issue**: This issue is good for first time contributors. If you've already contributed to Warehouse, please work on [another issue without this label](https://github.com/pypa/warehouse/issues?utf8=%E2%9C%93&q=is%3Aissue+is%3Aopen+-label%3A%22good+first+issue%22) instead. If there is not a corresponding pull request for this issue, it is up for grabs. For directions for getting set up, see our [Getting Started Guide](https://warehouse.pypa.io/development/getting-started/). If you are working on this issue and have questions, please feel free to ask them here, [`#pypa-dev` on Freenode](https://webchat.freenode.net/?channels=%23pypa-dev), or the [pypa-dev mailing list](https://groups.google.com/forum/#!forum/pypa-dev).
| I'm willing to work on this one. I'll let you know about the further progress @brainwane
Sounds good. @nitinprakash96 you go ahead and work on this one, and @saxenanurag can concentrate on #3185 instead. :) | 2018-04-03T07:31:35Z | [] | [] |
pypi/warehouse | 3,545 | pypi__warehouse-3545 | [
"3542"
] | 8ad7502d10d89689be20ab8f127f1a246dfd5f5f | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -718,12 +718,20 @@ def file_upload(request):
"Invalid or non-existent authentication information.",
)
- # distutils "helpfully" substitutes unknown, but "required" values with the
- # string "UNKNOWN". This is basically never what anyone actually wants so
- # we'll just go ahead and delete anything whose value is UNKNOWN.
+ # Do some cleanup of the various form fields
for key in list(request.POST):
- if str(request.POST.get(key)).strip() == "UNKNOWN":
- del request.POST[key]
+ value = request.POST.get(key)
+ if isinstance(value, str):
+ # distutils "helpfully" substitutes unknown, but "required" values
+ # with the string "UNKNOWN". This is basically never what anyone
+ # actually wants so we'll just go ahead and delete anything whose
+ # value is UNKNOWN.
+ if value.strip() == "UNKNOWN":
+ del request.POST[key]
+
+ # Escape NUL characters, which psycopg doesn't like
+ if '\x00' in value:
+ request.POST[key] = value.replace('\x00', '\\x00')
# We require protocol_version 1, it's the only supported version however
# passing a different version should raise an error.
| diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -1096,6 +1096,22 @@ def test_upload_cleans_unknown_values(
assert "name" not in db_request.POST
+ def test_upload_escapes_nul_characters(self, pyramid_config, db_request):
+ pyramid_config.testing_securitypolicy(userid=1)
+ db_request.POST = MultiDict({
+ "metadata_version": "1.2",
+ "name": "testing",
+ "summary": "I want to go to the \x00",
+ "version": "1.0",
+ "filetype": "sdist",
+ "md5_digest": "a fake md5 digest",
+ })
+
+ with pytest.raises(HTTPBadRequest):
+ legacy.file_upload(db_request)
+
+ assert "\x00" not in db_request.POST["summary"]
+
@pytest.mark.parametrize(
("has_signature", "digests"),
[
| Package upload fails with twine and setuptools
Attempting to add a new release with twine or setuptools results in a unhelpful `Received "500: Internal Server Error"`.
**OS:** Ubuntu 16.04.2 LTS
**Twine:** `twine version 1.11.0 (pkginfo: 1.4.2, requests: 2.18.4, setuptools: 39.0.1,
requests-toolbelt: 0.8.0, tqdm: 4.20.0)`
I have tried multiple repository URLs and configurations. The following is the last configuration and logs attempted:
**cat ~/.pypirc**
```
[distutils]
index-servers =
pypi
[pypi]
username: 0xgiddi
password: <redacted>
```
**twine upload dist/***
```Uploading distributions to https://upload.pypi.org/legacy/
Uploading pymbr-1.0.3-py2-none-any.whl
100%|████████████████████████████████████████████████████████████████████████████████████████████████████████████| 13.7k/13.7k [00:01<00:00, 8.43kB/s]
Received "500: Internal Server Error" Package upload appears to have failed. Retry 1 of 5
Uploading pymbr-1.0.3-py2-none-any.whl
100%|████████████████████████████████████████████████████████████████████████████████████████████████████████████| 13.7k/13.7k [00:01<00:00, 11.5kB/s]
Received "500: Internal Server Error" Package upload appears to have failed. Retry 2 of 5
Uploading pymbr-1.0.3-py2-none-any.whl
100%|████████████████████████████████████████████████████████████████████████████████████████████████████████████| 13.7k/13.7k [00:00<00:00, 16.2kB/s]
Received "500: Internal Server Error" Package upload appears to have failed. Retry 3 of 5
Uploading pymbr-1.0.3-py2-none-any.whl
100%|████████████████████████████████████████████████████████████████████████████████████████████████████████████| 13.7k/13.7k [00:00<00:00, 16.9kB/s]
Received "500: Internal Server Error" Package upload appears to have failed. Retry 4 of 5
Uploading pymbr-1.0.3-py2-none-any.whl
100%|████████████████████████████████████████████████████████████████████████████████████████████████████████████| 13.7k/13.7k [00:00<00:00, 18.2kB/s]
Received "500: Internal Server Error" Package upload appears to have failed. Retry 5 of 5
HTTPError: 500 Server Error: Internal Server Error for url: https://upload.pypi.org/legacy/
```
For fun, here is the log of a classic `python setup.py sdist upload`
```
running sdist
running egg_info
writing pymbr.egg-info/PKG-INFO
writing top-level names to pymbr.egg-info/top_level.txt
writing dependency_links to pymbr.egg-info/dependency_links.txt
reading manifest file 'pymbr.egg-info/SOURCES.txt'
writing manifest file 'pymbr.egg-info/SOURCES.txt'
running check
creating pymbr-1.0.3
creating pymbr-1.0.3/pymbr
creating pymbr-1.0.3/pymbr.egg-info
copying files to pymbr-1.0.3...
copying README.rst -> pymbr-1.0.3
copying setup.py -> pymbr-1.0.3
copying pymbr/__init__.py -> pymbr-1.0.3/pymbr
copying pymbr/bootcode.py -> pymbr-1.0.3/pymbr
copying pymbr/bootrecord.py -> pymbr-1.0.3/pymbr
copying pymbr/filesystem.py -> pymbr-1.0.3/pymbr
copying pymbr/partition.py -> pymbr-1.0.3/pymbr
copying pymbr.egg-info/PKG-INFO -> pymbr-1.0.3/pymbr.egg-info
copying pymbr.egg-info/SOURCES.txt -> pymbr-1.0.3/pymbr.egg-info
copying pymbr.egg-info/dependency_links.txt -> pymbr-1.0.3/pymbr.egg-info
copying pymbr.egg-info/top_level.txt -> pymbr-1.0.3/pymbr.egg-info
Writing pymbr-1.0.3/setup.cfg
Creating tar archive
removing 'pymbr-1.0.3' (and everything under it)
running upload
Submitting dist/pymbr-1.0.3.tar.gz to https://upload.pypi.org/legacy/
Upload failed (500): Internal Server Error
error: Upload failed (500): Internal Server Error
```
Package source: https://github.com/0xGiddi/pymbr
| @0xGiddi Could you upload, link, or email me ([email protected]) the `pymbr-1.0.3.tar.gz` file you're trying to upload?
@di Here you go:
[pymbr-1.0.3.tar.gz](https://github.com/pypa/warehouse/files/1873528/pymbr-1.0.3.tar.gz)
Interesting, this seems to be caused by your PyPI username starting with `0x`. Investigating... | 2018-04-04T01:30:17Z | [] | [] |
pypi/warehouse | 3,561 | pypi__warehouse-3561 | [
"778"
] | 78cd8a96b95b76e2039d8a2b77ff2aeff8c579f3 | diff --git a/warehouse/cache/origin/__init__.py b/warehouse/cache/origin/__init__.py
--- a/warehouse/cache/origin/__init__.py
+++ b/warehouse/cache/origin/__init__.py
@@ -18,6 +18,7 @@
from sqlalchemy.orm.session import Session
from warehouse import db
+from warehouse.cache.origin.derivers import html_cache_deriver
from warehouse.cache.origin.interfaces import IOriginCache
@@ -75,7 +76,7 @@ def wrapped(context, request):
request.add_response_callback(
functools.partial(
cacher.cache,
- sorted(context_keys + keys),
+ context_keys + keys,
seconds=seconds,
stale_while_revalidate=stale_while_revalidate,
stale_if_error=stale_if_error,
@@ -152,6 +153,7 @@ def includeme(config):
cache_class.create_service,
IOriginCache,
)
+ config.add_view_deriver(html_cache_deriver)
config.add_directive(
"register_origin_cache_keys",
diff --git a/warehouse/cache/origin/derivers.py b/warehouse/cache/origin/derivers.py
new file mode 100644
--- /dev/null
+++ b/warehouse/cache/origin/derivers.py
@@ -0,0 +1,35 @@
+# Licensed under the Apache License, Version 2.0 (the "License");
+# you may not use this file except in compliance with the License.
+# You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing, software
+# distributed under the License is distributed on an "AS IS" BASIS,
+# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+# See the License for the specific language governing permissions and
+# limitations under the License.
+
+import functools
+
+from warehouse.cache.origin.interfaces import IOriginCache
+
+
+def html_cache_deriver(view, info):
+ renderer = info.options.get('renderer')
+ if renderer and renderer.name.endswith('.html'):
+ def wrapper_view(context, request):
+ try:
+ cacher = request.find_service(IOriginCache)
+ except ValueError:
+ pass
+ else:
+ request.add_response_callback(
+ functools.partial(
+ cacher.cache,
+ ['all-html', renderer.name],
+ )
+ )
+ return view(context, request)
+ return wrapper_view
+ return view
diff --git a/warehouse/cache/origin/fastly.py b/warehouse/cache/origin/fastly.py
--- a/warehouse/cache/origin/fastly.py
+++ b/warehouse/cache/origin/fastly.py
@@ -11,6 +11,7 @@
# limitations under the License.
import urllib.parse
+import weakref
import requests
@@ -45,6 +46,7 @@ def __init__(self, *, api_key, service_id, purger):
self.api_key = api_key
self.service_id = service_id
self._purger = purger
+ self.keys = weakref.WeakKeyDictionary()
@classmethod
def create_service(cls, context, request):
@@ -56,7 +58,15 @@ def create_service(cls, context, request):
def cache(self, keys, request, response, *, seconds=None,
stale_while_revalidate=None, stale_if_error=None):
- response.headers["Surrogate-Key"] = " ".join(keys)
+
+ if request in self.keys:
+ self.keys[request].update(keys)
+ else:
+ self.keys[request] = set(keys)
+
+ response.headers["Surrogate-Key"] = " ".join(
+ sorted(self.keys[request])
+ )
values = []
| diff --git a/tests/unit/cache/origin/test_derivers.py b/tests/unit/cache/origin/test_derivers.py
new file mode 100644
--- /dev/null
+++ b/tests/unit/cache/origin/test_derivers.py
@@ -0,0 +1,89 @@
+# Licensed under the Apache License, Version 2.0 (the "License");
+# you may not use this file except in compliance with the License.
+# You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing, software
+# distributed under the License is distributed on an "AS IS" BASIS,
+# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+# See the License for the specific language governing permissions and
+# limitations under the License.
+
+import pretend
+
+from warehouse.cache.origin.derivers import html_cache_deriver
+
+
+def test_no_renderer():
+ view = pretend.stub()
+ info = pretend.stub(options={})
+
+ assert html_cache_deriver(view, info) == view
+
+
+def test_non_html_renderer():
+ view = pretend.stub()
+ renderer = pretend.stub(name='foo.txt')
+ info = pretend.stub(options={'renderer': renderer})
+
+ assert html_cache_deriver(view, info) == view
+
+
+def test_no_origin_cache_found():
+ view_result = pretend.stub()
+ view = pretend.call_recorder(lambda context, request: view_result)
+ renderer = pretend.stub(name='foo.html')
+ info = pretend.stub(options={'renderer': renderer})
+ context = pretend.stub()
+
+ def raise_valueerror(*a):
+ raise ValueError
+
+ request = pretend.stub(
+ find_service=raise_valueerror,
+ add_response_callback=pretend.call_recorder(lambda a: None)
+ )
+
+ assert html_cache_deriver(view, info)(context, request) == view_result
+ assert request.add_response_callback.calls == []
+
+
+def test_response_hook():
+
+ class Cache:
+
+ @staticmethod
+ @pretend.call_recorder
+ def cache(keys, request, response):
+ pass
+
+ response = pretend.stub()
+
+ @pretend.call_recorder
+ def view(context, request):
+ return response
+
+ context = pretend.stub()
+ cacher = Cache()
+ callbacks = []
+ request = pretend.stub(
+ find_service=lambda iface: cacher,
+ add_response_callback=callbacks.append,
+ )
+ info = pretend.stub(options={'renderer': pretend.stub(name='foo.html')})
+ derived_view = html_cache_deriver(view, info)
+
+ assert derived_view(context, request) is response
+ assert view.calls == [pretend.call(context, request)]
+ assert len(callbacks) == 1
+
+ callbacks[0](request, response)
+
+ assert cacher.cache.calls == [
+ pretend.call(
+ ['all-html', 'foo.html'],
+ request,
+ response,
+ ),
+ ]
diff --git a/tests/unit/cache/origin/test_fastly.py b/tests/unit/cache/origin/test_fastly.py
--- a/tests/unit/cache/origin/test_fastly.py
+++ b/tests/unit/cache/origin/test_fastly.py
@@ -138,6 +138,35 @@ def test_adds_surrogate_control(self):
),
}
+ def test_multiple_calls_to_cache_dont_overwrite_surrogate_keys(self):
+ request = pretend.stub()
+ response = pretend.stub(headers={})
+
+ cacher = fastly.FastlyCache(api_key=None, service_id=None, purger=None)
+ cacher.cache(["abc"], request, response)
+ cacher.cache(["defg"], request, response)
+
+ assert response.headers == {
+ "Surrogate-Key": "abc defg",
+ }
+
+ def test_multiple_calls_with_different_requests(self):
+ request_a = pretend.stub()
+ request_b = pretend.stub()
+ response_a = pretend.stub(headers={})
+ response_b = pretend.stub(headers={})
+
+ cacher = fastly.FastlyCache(api_key=None, service_id=None, purger=None)
+ cacher.cache(["abc"], request_a, response_a)
+ cacher.cache(["defg"], request_b, response_b)
+
+ assert response_a.headers == {
+ "Surrogate-Key": "abc",
+ }
+ assert response_b.headers == {
+ "Surrogate-Key": "defg",
+ }
+
def test_purge(self, monkeypatch):
purge_delay = pretend.call_recorder(lambda *a, **kw: None)
cacher = fastly.FastlyCache(
diff --git a/tests/unit/cache/origin/test_init.py b/tests/unit/cache/origin/test_init.py
--- a/tests/unit/cache/origin/test_init.py
+++ b/tests/unit/cache/origin/test_init.py
@@ -14,6 +14,7 @@
import pytest
from warehouse.cache import origin
+from warehouse.cache.origin.derivers import html_cache_deriver
from warehouse.cache.origin.interfaces import IOriginCache
@@ -189,7 +190,7 @@ def view(context, request):
assert cacher.cache.calls == [
pretend.call(
- sorted(["one", "two"] + ([] if keys is None else keys)),
+ ["one", "two"] + ([] if keys is None else keys),
request,
response,
seconds=seconds,
@@ -306,6 +307,7 @@ def test_includeme_with_origin_cache():
cache_class = pretend.stub(create_service=pretend.stub())
config = pretend.stub(
add_directive=pretend.call_recorder(lambda name, func: None),
+ add_view_deriver=pretend.call_recorder(lambda deriver: None),
registry=pretend.stub(
settings={
"origin_cache.backend":
@@ -324,6 +326,9 @@ def test_includeme_with_origin_cache():
origin.register_origin_cache_keys,
),
]
+ assert config.add_view_deriver.calls == [
+ pretend.call(html_cache_deriver),
+ ]
assert config.maybe_dotted.calls == [
pretend.call("warehouse.cache.origin.fastly.FastlyCache"),
]
| Document how to purge the HTTP cache when deploying the design
It'd be useful to document that you can do `curl -XPURGE https://warehouse.python.org/.../` when the design has been deployed to see the changes if they have been cached by Fastly.
| Another option we could maybe do here is tag all of the pages with which template(s) they depend on and whenever a deployment happens, do an automatic purge of any pages that had their templates changed. That will be more effort than just documenting the `curl -XPURGE` thing though.
| 2018-04-05T19:49:07Z | [] | [] |
pypi/warehouse | 3,568 | pypi__warehouse-3568 | [
"3221"
] | 57a8eca858965b027188e0955846ae2b61429b49 | diff --git a/warehouse/sessions.py b/warehouse/sessions.py
--- a/warehouse/sessions.py
+++ b/warehouse/sessions.py
@@ -263,6 +263,7 @@ def _process_response(self, request, response):
max_age=self.max_age,
httponly=True,
secure=request.scheme == "https",
+ samesite=b"lax"
)
| diff --git a/tests/unit/test_sessions.py b/tests/unit/test_sessions.py
--- a/tests/unit/test_sessions.py
+++ b/tests/unit/test_sessions.py
@@ -497,7 +497,7 @@ def test_invalidated_deletes_save_non_secure(self, monkeypatch,
)
response = pretend.stub(
set_cookie=pretend.call_recorder(
- lambda cookie, data, max_age, httponly, secure: None
+ lambda cookie, data, max_age, httponly, secure, samesite: None
)
)
session_factory._process_response(pyramid_request, response)
@@ -532,6 +532,7 @@ def test_invalidated_deletes_save_non_secure(self, monkeypatch,
max_age=12 * 60 * 60,
httponly=True,
secure=False,
+ samesite=b"lax",
),
]
| Set samesite=lax on session cookies
This is a strong defense-in-depth mechanism for protecting against CSRF. It's currently only respected by Chrome, but Firefox will add it as well.
| This may be blocked on https://github.com/Pylons/pyramid/issues/2733
Thanks, @alex.
Yeah, after Pyramid supports this, Warehouse should add it.
I think we're blocked on a WebOb release, not pyramid support.
I think this is unblocked now that #3554 has been merged. | 2018-04-05T22:57:24Z | [] | [] |
pypi/warehouse | 3,598 | pypi__warehouse-3598 | [
"3221"
] | f0e9641f5afec2362ccff9da3770df9fee6eba43 | diff --git a/warehouse/sessions.py b/warehouse/sessions.py
--- a/warehouse/sessions.py
+++ b/warehouse/sessions.py
@@ -263,7 +263,6 @@ def _process_response(self, request, response):
max_age=self.max_age,
httponly=True,
secure=request.scheme == "https",
- samesite=b"lax"
)
| diff --git a/requirements/tests.txt b/requirements/tests.txt
--- a/requirements/tests.txt
+++ b/requirements/tests.txt
@@ -224,9 +224,9 @@ urllib3==1.22 \
waitress==1.1.0 \
--hash=sha256:40b0f297a7f3af61fbfbdc67e59090c70dc150a1601c39ecc9f5f1d283fb931b \
--hash=sha256:d33cd3d62426c0f1b3cd84ee3d65779c7003aae3fc060dee60524d10a57f05a9
-WebOb==1.8.0 \
- --hash=sha256:ae809c05b667c3457a2937cdb4a7c7f07e90f26c651a340d37fdd1d5cf1fed27 \
- --hash=sha256:6fca7aa39bd2f6d2ff71f15a22223ff256c91f60b1ab52dac0ab38dc6ea9142f
+WebOb==1.7.4 \
+ --hash=sha256:63f4220492476c5c716b615baed7bf3d27040b3105014375787160dee0943115 \
+ --hash=sha256:8d10af182fda4b92193113ee1edeb687ab9dc44336b37d6804e413f0240d40d9
WebTest==2.0.29 \
--hash=sha256:9136514159a2e76a21751bf4ab5d3371e539c8ada8b950fcf68e307d9e584a07 \
--hash=sha256:dbbccc15ac2465066c95dc3a7de0d30cde3791e886ccbd7e91d5d2a2580c922d
diff --git a/tests/unit/test_sessions.py b/tests/unit/test_sessions.py
--- a/tests/unit/test_sessions.py
+++ b/tests/unit/test_sessions.py
@@ -497,7 +497,7 @@ def test_invalidated_deletes_save_non_secure(self, monkeypatch,
)
response = pretend.stub(
set_cookie=pretend.call_recorder(
- lambda cookie, data, max_age, httponly, secure, samesite: None
+ lambda cookie, data, max_age, httponly, secure: None
)
)
session_factory._process_response(pyramid_request, response)
@@ -532,7 +532,6 @@ def test_invalidated_deletes_save_non_secure(self, monkeypatch,
max_age=12 * 60 * 60,
httponly=True,
secure=False,
- samesite=b"lax",
),
]
diff --git a/tests/unit/utils/test_compression.py b/tests/unit/utils/test_compression.py
--- a/tests/unit/utils/test_compression.py
+++ b/tests/unit/utils/test_compression.py
@@ -14,7 +14,7 @@
import pytest
from pyramid.response import Response
-from webob.acceptparse import AcceptEncodingValidHeader, AcceptEncodingNoHeader
+from webob.acceptparse import Accept, NoAccept
from webob.response import gzip_app_iter
from warehouse.utils.compression import _compressor as compressor
@@ -54,7 +54,7 @@ def test_bails_if_content_encoding(self):
],
)
def test_sets_vary(self, vary, expected):
- request = pretend.stub(accept_encoding=AcceptEncodingNoHeader())
+ request = pretend.stub(accept_encoding=NoAccept())
response = Response(body=b"foo")
response.vary = vary
@@ -66,9 +66,7 @@ def test_compresses_non_streaming(self):
decompressed_body = b"foofoofoofoofoofoofoofoofoofoofoofoofoofoo"
compressed_body = b"".join(list(gzip_app_iter([decompressed_body])))
- request = pretend.stub(
- accept_encoding=AcceptEncodingValidHeader("gzip")
- )
+ request = pretend.stub(accept_encoding=Accept("gzip"))
response = Response(body=decompressed_body)
response.md5_etag()
@@ -85,9 +83,7 @@ def test_compresses_streaming(self):
decompressed_body = b"foofoofoofoofoofoofoofoofoofoofoofoofoofoo"
compressed_body = b"".join(list(gzip_app_iter([decompressed_body])))
- request = pretend.stub(
- accept_encoding=AcceptEncodingValidHeader("gzip")
- )
+ request = pretend.stub(accept_encoding=Accept("gzip"))
response = Response(app_iter=iter([decompressed_body]))
compressor(request, response)
@@ -100,9 +96,7 @@ def test_compresses_streaming_with_etag(self):
decompressed_body = b"foofoofoofoofoofoofoofoofoofoofoofoofoofoo"
compressed_body = b"".join(list(gzip_app_iter([decompressed_body])))
- request = pretend.stub(
- accept_encoding=AcceptEncodingValidHeader("gzip")
- )
+ request = pretend.stub(accept_encoding=Accept("gzip"))
response = Response(app_iter=iter([decompressed_body]))
response.etag = "foo"
@@ -117,9 +111,7 @@ def test_buffers_small_streaming(self):
decompressed_body = b"foofoofoofoofoofoofoofoofoofoofoofoofoofoo"
compressed_body = b"".join(list(gzip_app_iter([decompressed_body])))
- request = pretend.stub(
- accept_encoding=AcceptEncodingValidHeader("gzip")
- )
+ request = pretend.stub(accept_encoding=Accept("gzip"))
response = Response(
app_iter=iter([decompressed_body]),
content_length=len(decompressed_body),
@@ -132,9 +124,7 @@ def test_buffers_small_streaming(self):
assert response.body == compressed_body
def test_doesnt_compress_too_small(self):
- request = pretend.stub(
- accept_encoding=AcceptEncodingValidHeader("gzip")
- )
+ request = pretend.stub(accept_encoding=Accept("gzip"))
response = Response(body=b"foo")
compressor(request, response)
| Set samesite=lax on session cookies
This is a strong defense-in-depth mechanism for protecting against CSRF. It's currently only respected by Chrome, but Firefox will add it as well.
| This may be blocked on https://github.com/Pylons/pyramid/issues/2733
Thanks, @alex.
Yeah, after Pyramid supports this, Warehouse should add it.
I think we're blocked on a WebOb release, not pyramid support.
I think this is unblocked now that #3554 has been merged. | 2018-04-10T04:35:43Z | [] | [] |
pypi/warehouse | 3,600 | pypi__warehouse-3600 | [
"3221",
"3599"
] | f5723b0b50ab485a4cacee9799349d26124bcafc | diff --git a/warehouse/sessions.py b/warehouse/sessions.py
--- a/warehouse/sessions.py
+++ b/warehouse/sessions.py
@@ -263,6 +263,7 @@ def _process_response(self, request, response):
max_age=self.max_age,
httponly=True,
secure=request.scheme == "https",
+ samesite=b"lax"
)
| diff --git a/requirements/tests.txt b/requirements/tests.txt
--- a/requirements/tests.txt
+++ b/requirements/tests.txt
@@ -224,9 +224,9 @@ urllib3==1.22 \
waitress==1.1.0 \
--hash=sha256:40b0f297a7f3af61fbfbdc67e59090c70dc150a1601c39ecc9f5f1d283fb931b \
--hash=sha256:d33cd3d62426c0f1b3cd84ee3d65779c7003aae3fc060dee60524d10a57f05a9
-WebOb==1.7.4 \
- --hash=sha256:63f4220492476c5c716b615baed7bf3d27040b3105014375787160dee0943115 \
- --hash=sha256:8d10af182fda4b92193113ee1edeb687ab9dc44336b37d6804e413f0240d40d9
+WebOb==1.8.0 \
+ --hash=sha256:ae809c05b667c3457a2937cdb4a7c7f07e90f26c651a340d37fdd1d5cf1fed27 \
+ --hash=sha256:6fca7aa39bd2f6d2ff71f15a22223ff256c91f60b1ab52dac0ab38dc6ea9142f
WebTest==2.0.29 \
--hash=sha256:9136514159a2e76a21751bf4ab5d3371e539c8ada8b950fcf68e307d9e584a07 \
--hash=sha256:dbbccc15ac2465066c95dc3a7de0d30cde3791e886ccbd7e91d5d2a2580c922d
diff --git a/tests/unit/test_sessions.py b/tests/unit/test_sessions.py
--- a/tests/unit/test_sessions.py
+++ b/tests/unit/test_sessions.py
@@ -497,7 +497,7 @@ def test_invalidated_deletes_save_non_secure(self, monkeypatch,
)
response = pretend.stub(
set_cookie=pretend.call_recorder(
- lambda cookie, data, max_age, httponly, secure: None
+ lambda cookie, data, max_age, httponly, secure, samesite: None
)
)
session_factory._process_response(pyramid_request, response)
@@ -532,6 +532,7 @@ def test_invalidated_deletes_save_non_secure(self, monkeypatch,
max_age=12 * 60 * 60,
httponly=True,
secure=False,
+ samesite=b"lax",
),
]
diff --git a/tests/unit/utils/test_compression.py b/tests/unit/utils/test_compression.py
--- a/tests/unit/utils/test_compression.py
+++ b/tests/unit/utils/test_compression.py
@@ -14,7 +14,7 @@
import pytest
from pyramid.response import Response
-from webob.acceptparse import Accept, NoAccept
+from webob.acceptparse import AcceptEncodingValidHeader, AcceptEncodingNoHeader
from webob.response import gzip_app_iter
from warehouse.utils.compression import _compressor as compressor
@@ -54,7 +54,7 @@ def test_bails_if_content_encoding(self):
],
)
def test_sets_vary(self, vary, expected):
- request = pretend.stub(accept_encoding=NoAccept())
+ request = pretend.stub(accept_encoding=AcceptEncodingNoHeader())
response = Response(body=b"foo")
response.vary = vary
@@ -66,7 +66,9 @@ def test_compresses_non_streaming(self):
decompressed_body = b"foofoofoofoofoofoofoofoofoofoofoofoofoofoo"
compressed_body = b"".join(list(gzip_app_iter([decompressed_body])))
- request = pretend.stub(accept_encoding=Accept("gzip"))
+ request = pretend.stub(
+ accept_encoding=AcceptEncodingValidHeader("gzip")
+ )
response = Response(body=decompressed_body)
response.md5_etag()
@@ -83,7 +85,9 @@ def test_compresses_streaming(self):
decompressed_body = b"foofoofoofoofoofoofoofoofoofoofoofoofoofoo"
compressed_body = b"".join(list(gzip_app_iter([decompressed_body])))
- request = pretend.stub(accept_encoding=Accept("gzip"))
+ request = pretend.stub(
+ accept_encoding=AcceptEncodingValidHeader("gzip")
+ )
response = Response(app_iter=iter([decompressed_body]))
compressor(request, response)
@@ -96,7 +100,9 @@ def test_compresses_streaming_with_etag(self):
decompressed_body = b"foofoofoofoofoofoofoofoofoofoofoofoofoofoo"
compressed_body = b"".join(list(gzip_app_iter([decompressed_body])))
- request = pretend.stub(accept_encoding=Accept("gzip"))
+ request = pretend.stub(
+ accept_encoding=AcceptEncodingValidHeader("gzip")
+ )
response = Response(app_iter=iter([decompressed_body]))
response.etag = "foo"
@@ -111,7 +117,9 @@ def test_buffers_small_streaming(self):
decompressed_body = b"foofoofoofoofoofoofoofoofoofoofoofoofoofoo"
compressed_body = b"".join(list(gzip_app_iter([decompressed_body])))
- request = pretend.stub(accept_encoding=Accept("gzip"))
+ request = pretend.stub(
+ accept_encoding=AcceptEncodingValidHeader("gzip")
+ )
response = Response(
app_iter=iter([decompressed_body]),
content_length=len(decompressed_body),
@@ -124,7 +132,9 @@ def test_buffers_small_streaming(self):
assert response.body == compressed_body
def test_doesnt_compress_too_small(self):
- request = pretend.stub(accept_encoding=Accept("gzip"))
+ request = pretend.stub(
+ accept_encoding=AcceptEncodingValidHeader("gzip")
+ )
response = Response(body=b"foo")
compressor(request, response)
| Set samesite=lax on session cookies
This is a strong defense-in-depth mechanism for protecting against CSRF. It's currently only respected by Chrome, but Firefox will add it as well.
Update webob to 1.8.0
This PR updates [WebOb](https://pypi.org/project/WebOb) from **1.7.4** to **1.8.0**.
<details>
<summary>Changelog</summary>
### 1.8.0
```
------------------
Feature
~~~~~~~
- ``request.POST`` now supports any requests with the appropriate
Content-Type. Allowing any HTTP method to access form encoded content,
including DELETE, PUT, and others. See
https://github.com/Pylons/webob/pull/352
Compatibility
~~~~~~~~~~~~~
- WebOb is no longer officially supported on Python 3.3 which was EOL'ed on
2017-09-29.
Backwards Incompatibilities
~~~~~~~~~~~~~~~~~~~~~~~~~~~
- Many changes have been made to the way WebOb does Accept handling, not just
for the Accept header itself, but also for Accept-Charset, Accept-Encoding
and Accept-Language. This was a `Google Summer of Code
<https://developers.google.com/open-source/gsoc/>`_ project completed by
Whiteroses (https://github.com/whiteroses). Many thanks to Google for running
GSoC, the Python Software Foundation for organising and a huge thanks to Ira
for completing the work. See https://github.com/Pylons/webob/pull/338 and
https://github.com/Pylons/webob/pull/335. Documentation is available at
https://docs.pylonsproject.org/projects/webob/en/master/api/webob.html
- When calling a ``wsgify`` decorated function, the default arguments passed
to ``wsgify`` are now used when called with the request, and not as a
`start_response`
.. code::
def hello(req, name):
return "Hello, %s!" % name
app = wsgify(hello, args=("Fred",))
req = Request.blank('/')
resp = req.get_response(app) => "Hello, Fred"
resp2 = app(req) => "Hello, Fred"
Previously the ``resp2`` line would have failed with a ``TypeError``. With
this change there is no way to override the default arguments with no
arguments. See https://github.com/Pylons/webob/pull/203
- When setting ``app_iter`` on a ``Response`` object the ``content_md5`` header
is no longer cleared. This behaviour is odd and disallows setting the
``content_md5`` and then returning an iterator for chunked content encoded
responses. See https://github.com/Pylons/webob/issues/86
Experimental Features
~~~~~~~~~~~~~~~~~~~~~
These features are experimental and may change at any point in the future.
- The cookie APIs now have the ability to set the SameSite attribute on a
cookie in both ``webob.cookies.make_cookie`` and
``webob.cookies.CookieProfile``. See https://github.com/Pylons/webob/pull/255
Bugfix
~~~~~~
- Exceptions now use string.Template.safe_substitute rather than
string.Template.substitute. The latter would raise for missing mappings, the
former will simply not substitute the missing variable. This is safer in case
the WSGI environ does not contain the keys necessary for the body template.
See https://github.com/Pylons/webob/issues/345.
- Request.host_url, Request.host_port, Request.domain correctly parse IPv6 Host
headers as provided by a browser. See
https://github.com/Pylons/webob/pull/332
- Request.authorization would raise ValueError for unusual or malformed header
values. See https://github.com/Pylons/webob/issues/231
- Allow unnamed fields in form data to be properly transcoded when calling
request.decode with an alternate encoding. See
https://github.com/Pylons/webob/pull/309
- ``Response.__init__`` would discard ``app_iter`` when a ``Response`` had no
body, this would cause issues when ``app_iter`` was an object that was tied
to the life-cycle of a web application and had to be properly closed.
``app_iter`` is more advanced API for ``Response`` and thus even if it
contains a body and is thus against the HTTP RFC's, we should let the users
shoot themselves by returning a body. See
https://github.com/Pylons/webob/issues/305
```
</details>
<details>
<summary>Links</summary>
- PyPI: https://pypi.org/project/webob
- Changelog: https://pyup.io/changelogs/webob/
- Homepage: http://webob.org/
</details>
| This may be blocked on https://github.com/Pylons/pyramid/issues/2733
Thanks, @alex.
Yeah, after Pyramid supports this, Warehouse should add it.
I think we're blocked on a WebOb release, not pyramid support.
I think this is unblocked now that #3554 has been merged.
| 2018-04-10T04:51:50Z | [] | [] |
pypi/warehouse | 3,601 | pypi__warehouse-3601 | [
"3578"
] | f386666fe18384f8f3dd899e2ed86724342d1527 | diff --git a/warehouse/forklift/__init__.py b/warehouse/forklift/__init__.py
--- a/warehouse/forklift/__init__.py
+++ b/warehouse/forklift/__init__.py
@@ -15,6 +15,11 @@
# project.
+def _help_url(request, **kwargs):
+ warehouse_domain = request.registry.settings.get("warehouse.domain")
+ return request.route_url('help', _host=warehouse_domain, **kwargs)
+
+
def includeme(config):
# We need to get the value of the Warehouse and Forklift domains, we'll use
# these to segregate the Warehouse routes from the Forklift routes until
@@ -45,6 +50,7 @@ def includeme(config):
"doc_upload",
domain=forklift,
)
+ config.add_request_method(_help_url, name="help_url")
if forklift:
config.add_template_view(
diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -820,8 +820,8 @@ def file_upload(request):
("New Project Registration Temporarily Disabled "
"See {projecthelp} for details")
.format(
- projecthelp=request.route_url(
- 'help', _anchor='admin-intervention')),
+ projecthelp=request.help_url(_anchor='admin-intervention'),
+ ),
) from None
# Ensure that user has at least one verified email address. This should
@@ -835,10 +835,9 @@ def file_upload(request):
"please verify at least one address before registering "
"a new project on PyPI. See {projecthelp} "
"for more information.").format(
- request.user.username,
- projecthelp=request.route_url(
- 'help', _anchor='verified-email'
- )),
+ request.user.username,
+ projecthelp=request.help_url(_anchor='verified-email'),
+ ),
) from None
# Before we create the project, we're going to check our blacklist to
@@ -853,8 +852,8 @@ def file_upload(request):
"See {projecthelp} "
"for more information.").format(
name=form.name.data,
- projecthelp=request.route_url(
- 'help', _anchor='project-name')),
+ projecthelp=request.help_url(_anchor='project-name'),
+ ),
) from None
# Also check for collisions with Python Standard Library modules.
@@ -865,9 +864,9 @@ def file_upload(request):
("The name {name!r} is not allowed (conflict with Python "
"Standard Library module name). See "
"{projecthelp} for more information.").format(
- name=form.name.data,
- projecthelp=request.route_url(
- 'help', _anchor='project-name')),
+ name=form.name.data,
+ projecthelp=request.help_url(_anchor='project-name')
+ )
) from None
# The project doesn't exist in our database, so we'll add it along with
@@ -905,8 +904,10 @@ def file_upload(request):
HTTPForbidden,
("The user '{0}' is not allowed to upload to project '{1}'. "
"See {2} for more information.")
- .format(request.user.username, project.name, request.route_url(
- 'help', _anchor='project-name')
+ .format(
+ request.user.username,
+ project.name,
+ request.help_url(_anchor='project-name')
)
)
@@ -1073,9 +1074,7 @@ def file_upload(request):
name=project.name,
limit=file_size_limit // (1024 * 1024)) +
"See " +
- request.route_url(
- 'help', _anchor='file-size-limit'
- ),
+ request.help_url(_anchor='file-size-limit'),
)
fp.write(chunk)
for hasher in file_hashes.values():
@@ -1118,9 +1117,7 @@ def file_upload(request):
# ref: https://github.com/pypa/warehouse/issues/3482
# ref: https://github.com/pypa/twine/issues/332
"File already exists. See " +
- request.route_url(
- 'help', _anchor='file-name-reuse'
- )
+ request.help_url(_anchor='file-name-reuse')
)
# Check to see if the file that was uploaded exists in our filename log
@@ -1132,9 +1129,7 @@ def file_upload(request):
HTTPBadRequest,
"This filename has previously been used, you should use a "
"different version. "
- "See " + request.route_url(
- 'help', _anchor='file-name-reuse'
- ),
+ "See " + request.help_url(_anchor='file-name-reuse'),
)
# Check to see if uploading this file would create a duplicate sdist
| diff --git a/tests/unit/forklift/test_init.py b/tests/unit/forklift/test_init.py
--- a/tests/unit/forklift/test_init.py
+++ b/tests/unit/forklift/test_init.py
@@ -13,51 +13,84 @@
import pretend
import pytest
-from warehouse.forklift import includeme
+from warehouse import forklift
[email protected]("forklift", [None, "upload.pypi.io"])
-def test_includeme(forklift):
[email protected]("forklift_domain", [None, "upload.pypi.io"])
+def test_includeme(forklift_domain, monkeypatch):
settings = {}
- if forklift:
- settings["forklift.domain"] = forklift
+ if forklift_domain:
+ settings["forklift.domain"] = forklift_domain
+
+ _help_url = pretend.stub()
+ monkeypatch.setattr(forklift, '_help_url', _help_url)
config = pretend.stub(
get_settings=lambda: settings,
include=pretend.call_recorder(lambda n: None),
add_legacy_action_route=pretend.call_recorder(lambda *a, **k: None),
add_template_view=pretend.call_recorder(lambda *a, **kw: None),
+ add_request_method=pretend.call_recorder(lambda *a, **kw: None),
)
- includeme(config)
+ forklift.includeme(config)
- config.include.calls == [pretend.call(".action_routing")]
- config.add_legacy_action_route.calls == [
+ assert config.include.calls == [pretend.call(".action_routing")]
+ assert config.add_legacy_action_route.calls == [
pretend.call(
"forklift.legacy.file_upload",
"file_upload",
- domain=forklift,
+ domain=forklift_domain,
+ ),
+ pretend.call(
+ "forklift.legacy.submit",
+ "submit",
+ domain=forklift_domain,
),
- pretend.call("forklift.legacy.submit", "submit", domain=forklift),
pretend.call(
"forklift.legacy.submit_pkg_info",
"submit_pkg_info",
- domain=forklift,
+ domain=forklift_domain,
),
pretend.call(
"forklift.legacy.doc_upload",
"doc_upload",
- domain=forklift,
+ domain=forklift_domain,
),
]
- if forklift:
- config.add_template_view.calls == [
+ assert config.add_request_method.calls == [
+ pretend.call(_help_url, name='help_url'),
+ ]
+ if forklift_domain:
+ assert config.add_template_view.calls == [
pretend.call(
"forklift.index",
"/",
"upload.html",
- route_kw={"domain": forklift},
+ route_kw={"domain": forklift_domain},
+ ),
+ pretend.call(
+ 'forklift.legacy.invalid_request',
+ '/legacy/',
+ 'upload.html',
+ route_kw={'domain': 'upload.pypi.io'},
),
]
else:
- config.add_template_view.calls == []
+ assert config.add_template_view.calls == []
+
+
+def test_help_url():
+ warehouse_domain = pretend.stub()
+ result = pretend.stub()
+ request = pretend.stub(
+ route_url=pretend.call_recorder(lambda *a, **kw: result),
+ registry=pretend.stub(
+ settings={'warehouse.domain': warehouse_domain},
+ ),
+ )
+
+ assert forklift._help_url(request, _anchor='foo') == result
+ assert request.route_url.calls == [
+ pretend.call('help', _host=warehouse_domain, _anchor='foo'),
+ ]
diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -959,8 +959,8 @@ def test_fails_with_invalid_names(self, pyramid_config, db_request, name):
),
})
- db_request.route_url = pretend.call_recorder(
- lambda route, **kw: "/the/help/url/"
+ db_request.help_url = pretend.call_recorder(
+ lambda **kw: "/the/help/url/"
)
with pytest.raises(HTTPBadRequest) as excinfo:
@@ -968,8 +968,8 @@ def test_fails_with_invalid_names(self, pyramid_config, db_request, name):
resp = excinfo.value
- assert db_request.route_url.calls == [
- pretend.call('help', _anchor='project-name')
+ assert db_request.help_url.calls == [
+ pretend.call(_anchor='project-name')
]
assert resp.status_code == 400
@@ -1005,8 +1005,8 @@ def test_fails_with_stdlib_names(self, pyramid_config, db_request, name):
),
})
- db_request.route_url = pretend.call_recorder(
- lambda route, **kw: "/the/help/url/"
+ db_request.help_url = pretend.call_recorder(
+ lambda **kw: "/the/help/url/"
)
with pytest.raises(HTTPBadRequest) as excinfo:
@@ -1014,8 +1014,8 @@ def test_fails_with_stdlib_names(self, pyramid_config, db_request, name):
resp = excinfo.value
- assert db_request.route_url.calls == [
- pretend.call('help', _anchor='project-name')
+ assert db_request.help_url.calls == [
+ pretend.call(_anchor='project-name')
]
assert resp.status_code == 400
@@ -1045,8 +1045,8 @@ def test_fails_with_admin_flag_set(self, pyramid_config, db_request):
),
})
- db_request.route_url = pretend.call_recorder(
- lambda route, **kw: "/the/help/url/"
+ db_request.help_url = pretend.call_recorder(
+ lambda **kw: "/the/help/url/"
)
with pytest.raises(HTTPForbidden) as excinfo:
@@ -1650,8 +1650,8 @@ def test_upload_fails_with_too_large_file(self, pyramid_config,
type="application/tar",
),
})
- db_request.route_url = pretend.call_recorder(
- lambda route, **kw: "/the/help/url/"
+ db_request.help_url = pretend.call_recorder(
+ lambda **kw: "/the/help/url/"
)
with pytest.raises(HTTPBadRequest) as excinfo:
@@ -1659,8 +1659,8 @@ def test_upload_fails_with_too_large_file(self, pyramid_config,
resp = excinfo.value
- assert db_request.route_url.calls == [
- pretend.call('help', _anchor='file-size-limit')
+ assert db_request.help_url.calls == [
+ pretend.call(_anchor='file-size-limit')
]
assert resp.status_code == 400
assert resp.status == (
@@ -1732,8 +1732,8 @@ def test_upload_fails_with_previously_used_filename(self, pyramid_config,
})
db_request.db.add(Filename(filename=filename))
- db_request.route_url = pretend.call_recorder(
- lambda route, **kw: "/the/help/url/"
+ db_request.help_url = pretend.call_recorder(
+ lambda **kw: "/the/help/url/"
)
with pytest.raises(HTTPBadRequest) as excinfo:
@@ -1741,8 +1741,8 @@ def test_upload_fails_with_previously_used_filename(self, pyramid_config,
resp = excinfo.value
- assert db_request.route_url.calls == [
- pretend.call('help', _anchor='file-name-reuse')
+ assert db_request.help_url.calls == [
+ pretend.call(_anchor='file-name-reuse'),
]
assert resp.status_code == 400
assert resp.status == (
@@ -1846,16 +1846,16 @@ def test_upload_fails_with_existing_filename_diff_content(self,
),
),
)
- db_request.route_url = pretend.call_recorder(
- lambda route, **kw: "/the/help/url/"
+ db_request.help_url = pretend.call_recorder(
+ lambda **kw: "/the/help/url/"
)
with pytest.raises(HTTPBadRequest) as excinfo:
legacy.file_upload(db_request)
resp = excinfo.value
- assert db_request.route_url.calls == [
- pretend.call('help', _anchor='file-name-reuse')
+ assert db_request.help_url.calls == [
+ pretend.call(_anchor='file-name-reuse')
]
assert resp.status_code == 400
assert resp.status == "400 File already exists. See /the/help/url/"
@@ -1904,8 +1904,8 @@ def test_upload_fails_with_diff_filename_same_blake2(self,
),
),
)
- db_request.route_url = pretend.call_recorder(
- lambda route, **kw: "/the/help/url/"
+ db_request.help_url = pretend.call_recorder(
+ lambda **kw: "/the/help/url/"
)
with pytest.raises(HTTPBadRequest) as excinfo:
@@ -1913,8 +1913,8 @@ def test_upload_fails_with_diff_filename_same_blake2(self,
resp = excinfo.value
- assert db_request.route_url.calls == [
- pretend.call('help', _anchor='file-name-reuse')
+ assert db_request.help_url.calls == [
+ pretend.call(_anchor='file-name-reuse')
]
assert resp.status_code == 400
assert resp.status == "400 File already exists. See /the/help/url/"
@@ -2058,8 +2058,8 @@ def test_upload_fails_without_permission(self, pyramid_config, db_request):
),
})
- db_request.route_url = pretend.call_recorder(
- lambda route, **kw: "/the/help/url/"
+ db_request.help_url = pretend.call_recorder(
+ lambda **kw: "/the/help/url/"
)
with pytest.raises(HTTPForbidden) as excinfo:
@@ -2067,8 +2067,8 @@ def test_upload_fails_without_permission(self, pyramid_config, db_request):
resp = excinfo.value
- assert db_request.route_url.calls == [
- pretend.call('help', _anchor='project-name')
+ assert db_request.help_url.calls == [
+ pretend.call(_anchor='project-name')
]
assert resp.status_code == 403
assert resp.status == (
@@ -2762,8 +2762,8 @@ def test_upload_requires_verified_email(self, pyramid_config, db_request,
resp = legacy.file_upload(db_request)
assert resp.status_code == 200
else:
- db_request.route_url = pretend.call_recorder(
- lambda route, **kw: "/the/help/url/"
+ db_request.help_url = pretend.call_recorder(
+ lambda **kw: "/the/help/url/"
)
with pytest.raises(HTTPBadRequest) as excinfo:
@@ -2771,8 +2771,8 @@ def test_upload_requires_verified_email(self, pyramid_config, db_request,
resp = excinfo.value
- assert db_request.route_url.calls == [
- pretend.call('help', _anchor='verified-email')
+ assert db_request.help_url.calls == [
+ pretend.call(_anchor='verified-email')
]
assert resp.status_code == 400
assert resp.status == (
| "no verified email addresses" error message generates wrong URL for pypi.org help
I'm trying to upload a package using `python3 setup.py sdist upload`. It fails:
```
error: Upload failed (400): User 'whitequark' has no verified email addresses, please verify at least one address before registering a new project on PyPI. See https://upload.pypi.org/help/#verified-email for more information.
```
Sure. The document it links to says:
> You can manage your account's email addresses in your [Profile](https://upload.pypi.org/manage/account/). This also allows for sending a new confirmation email for users who signed up in the past, before we began enforcing this policy.
The Profile link is broken and leads to a 404 page.
Sure. Maybe I could reset my password instead. The help page says:
> You should be able to [reset your password](https://upload.pypi.org/account/reset-password/) on the site; you'll receive an email with a password reset link.
The reset password link is also broken and leads to a 404 page.
In addition there are no account management functions whatsoever linked from anywhere on [upload.pypi.org](https://upload.pypi.org).
| Ah, the link to Profile should be to pypi.org, not upload.pypi.org.
That worked, thanks!
Looks like this is a bug in how we're generating URLs in our error messages. Thanks for the report!
~I think the URL in the error is fine? At least, it correctly leads me to the help page. It's the others that return 404.~ Nevermind, I just figured out that https://pypi.org/help/#verified-email has the correct URLs.
The help page is actually at https://pypi.org/help/, the upload domain shouldn't be displaying it.
This affected another user: https://github.com/pypa/packaging-problems/issues/137#issuecomment-379971088 | 2018-04-10T06:19:19Z | [] | [] |
pypi/warehouse | 3,647 | pypi__warehouse-3647 | [
"3643"
] | 764ac4b5384249fad034d0b8512d559822e88317 | diff --git a/warehouse/packaging/models.py b/warehouse/packaging/models.py
--- a/warehouse/packaging/models.py
+++ b/warehouse/packaging/models.py
@@ -437,7 +437,8 @@ def urls(self):
def github_repo_info_url(self):
for parsed in [urlparse(url) for url in self.urls.values()]:
segments = parsed.path.strip('/').rstrip('/').split('/')
- if parsed.netloc == 'github.com' and len(segments) >= 2:
+ if (parsed.netloc == 'github.com' or
+ parsed.netloc == 'www.github.com') and len(segments) >= 2:
user_name, repo_name = segments[:2]
return f"https://api.github.com/repos/{user_name}/{repo_name}"
| diff --git a/tests/unit/packaging/test_models.py b/tests/unit/packaging/test_models.py
--- a/tests/unit/packaging/test_models.py
+++ b/tests/unit/packaging/test_models.py
@@ -305,6 +305,10 @@ def test_acl(self, db_session):
"https://github.com/pypa/warehouse/tree/master",
"https://api.github.com/repos/pypa/warehouse"
),
+ (
+ "https://www.github.com/pypa/warehouse",
+ "https://api.github.com/repos/pypa/warehouse"
+ ),
(
"https://github.com/pypa/",
None
| Automagic GitHub repo stats display doesn't find www.github.com/user/repo URLs
As noted [here](https://github.com/pypa/warehouse/pull/2163#pullrequestreview-111691465), the automagic sidebar stats feature when a GitHub repo is the project homepage doesn't activate if the URL is written using `www.github.com`; it only finds `github.com` URLs.
| We probably should make sure that this works for URLs like `github.com` and `www.github.com`, but not something like `thisisnotgithub.com`.
---
**Good First Issue**: This issue is good for first time contributors. If you've already contributed to Warehouse, please work on [another issue without this label](https://github.com/pypa/warehouse/issues?utf8=%E2%9C%93&q=is%3Aissue+is%3Aopen+-label%3A%22good+first+issue%22) instead. If there is not a corresponding pull request for this issue, it is up for grabs. For directions for getting set up, see our [Getting Started Guide](https://warehouse.pypa.io/development/getting-started/). If you are working on this issue and have questions, please feel free to ask them here, [`#pypa-dev` on Freenode](https://webchat.freenode.net/?channels=%23pypa-dev), or the [pypa-dev mailing list](https://groups.google.com/forum/#!forum/pypa-dev).
Mm, <nod>... `.endswith('.github.com')` might suffice?
> Mm, ... `.endswith('.github.com')` might suffice?
I don't think this would work for a URL like `https://github.com/pypa/warehouse`
Indeed. Breaky-breaky. Might not be able to avoid a compound condition?
if (parsed.netloc == 'github.com' or parsed.netloc.endswith('.github.com')) and len(segments) >= 2:
Are there any other common `*.github.com` URLs that would ***not*** be composed as `*.github.com/user/repo/...`, and that someone might want to use as a homepage URL?
If so, a pattern matching condition might get pretty messy. A narrower compound condition might be better.
if (parsed.netloc == 'github.com' or parsed.netloc == 'www.github.com') and ...
@bskinn Hope you don't mind -- since this is an improvement to a new feature, I'm marking it as something we can do after we shut down the legacy PyPI site.
@brainwane Nope! I figured it would get slotted for further down the pipeline, since it's a minor glitch.
To be clear: if you made a PR to fix this I would merge it, it's just not going to get fixed by one of the maintainers soon.
@di I can put together a PR to fix it, no problem; but I'm not set up with docker &c. to run the test suite locally. Can I just rely on Travis to test it for me?
~Also, if I'd need to add a test for the new behavior, I'd need guidance as to where in the test suite to add it.~ Nevermind, [found the current test](https://github.com/pypa/warehouse/blob/a4ca4b580d74c68afe082ca6cff05c9e92ca1865/tests/unit/packaging/test_models.py#L292-L330).
@bskinn Travis will definitely test it for you. You probably don't need to be able to run the tests locally to fix this issue, but it's not too complicated and we have a pretty good guide: https://warehouse.readthedocs.io/development/getting-started/ | 2018-04-12T20:30:15Z | [] | [] |
pypi/warehouse | 3,676 | pypi__warehouse-3676 | [
"3674"
] | 5b01b48de957ef6bf433d895a85068fd847f1773 | diff --git a/warehouse/packaging/search.py b/warehouse/packaging/search.py
--- a/warehouse/packaging/search.py
+++ b/warehouse/packaging/search.py
@@ -55,11 +55,11 @@ class Meta:
@classmethod
def from_db(cls, release):
- obj = cls(meta={"id": release.project.normalized_name})
- obj["name"] = release.project.name
- obj["normalized_name"] = release.project.normalized_name
- obj["version"] = [r.version for r in release.project.all_versions]
- obj["latest_version"] = release.project.latest_version.version
+ obj = cls(meta={"id": release.normalized_name})
+ obj["name"] = release.name
+ obj["normalized_name"] = release.normalized_name
+ obj["version"] = release.all_versions
+ obj["latest_version"] = release.latest_version
obj["summary"] = release.summary
obj["description"] = release.description
obj["author"] = release.author
@@ -71,6 +71,6 @@ def from_db(cls, release):
obj["keywords"] = release.keywords
obj["platform"] = release.platform
obj["created"] = release.created
- obj["classifiers"] = list(release.classifiers)
+ obj["classifiers"] = release.classifiers
return obj
diff --git a/warehouse/search/tasks.py b/warehouse/search/tasks.py
--- a/warehouse/search/tasks.py
+++ b/warehouse/search/tasks.py
@@ -14,10 +14,11 @@
import os
from elasticsearch.helpers import parallel_bulk
-from sqlalchemy import and_
-from sqlalchemy.orm import lazyload, joinedload, load_only
+from sqlalchemy import and_, func
+from sqlalchemy.orm import aliased
-from warehouse.packaging.models import Release
+from warehouse.packaging.models import (
+ Classifier, Project, Release, release_classifiers)
from warehouse.packaging.search import Project as ProjectDocType
from warehouse.search.utils import get_index
from warehouse import tasks
@@ -27,30 +28,63 @@
def _project_docs(db):
releases_list = (
- db.query(Release)
- .options(load_only(
- "name", "version"))
- .distinct(Release.name)
- .order_by(
- Release.name,
- Release.is_prerelease.nullslast(),
- Release._pypi_ordering.desc())
- ).subquery('release_list')
+ db.query(Release.name, Release.version)
+ .order_by(
+ Release.name,
+ Release.is_prerelease.nullslast(),
+ Release._pypi_ordering.desc(),
+ )
+ .distinct(Release.name)
+ .subquery("release_list")
+ )
+
+ r = aliased(Release, name="r")
+
+ all_versions = (
+ db.query(func.array_agg(r.version))
+ .filter(r.name == Release.name)
+ .correlate(Release)
+ .as_scalar()
+ .label("all_versions")
+ )
+
+ classifiers = (
+ db.query(func.array_agg(Classifier.classifier))
+ .select_from(release_classifiers)
+ .join(Classifier, Classifier.id == release_classifiers.c.trove_id)
+ .filter(Release.name == release_classifiers.c.name)
+ .filter(Release.version == release_classifiers.c.version)
+ .correlate(Release)
+ .as_scalar()
+ .label("classifiers")
+ )
release_data = (
- db.query(Release)
- .options(load_only(
- "summary", "description", "author",
- "author_email", "maintainer", "maintainer_email",
- "home_page", "download_url", "keywords", "platform",
- "created"))
- .options(lazyload("*"),
- (joinedload(Release.project)
- .load_only("normalized_name", "name")),
- joinedload(Release._classifiers).load_only("classifier"))
- .filter(and_(
- Release.name == releases_list.c.name,
- Release.version == releases_list.c.version))
+ db.query(
+ Release.description,
+ Release.name,
+ Release.version.label("latest_version"),
+ all_versions,
+ Release.author,
+ Release.author_email,
+ Release.maintainer,
+ Release.maintainer_email,
+ Release.home_page,
+ Release.summary,
+ Release.keywords,
+ Release.platform,
+ Release.download_url,
+ Release.created,
+ classifiers,
+ Project.normalized_name,
+ Project.name,
+ )
+ .select_from(releases_list)
+ .join(Release, and_(
+ Release.name == releases_list.c.name,
+ Release.version == releases_list.c.version))
+ .outerjoin(Release.project)
+ .order_by(Release.name)
)
for release in windowed_query(release_data, Release.name, 1000):
| diff --git a/tests/unit/packaging/test_search.py b/tests/unit/packaging/test_search.py
--- a/tests/unit/packaging/test_search.py
+++ b/tests/unit/packaging/test_search.py
@@ -18,18 +18,16 @@
def test_build_search():
release = pretend.stub(
- project=pretend.stub(
- name="Foobar",
- normalized_name="foobar",
- all_versions=[
- pretend.stub(version="5.0.dev0"),
- pretend.stub(version="4.0"),
- pretend.stub(version="3.0"),
- pretend.stub(version="2.0"),
- pretend.stub(version="1.0"),
- ],
- latest_version=pretend.stub(version="4.0"),
- ),
+ name="Foobar",
+ normalized_name="foobar",
+ all_versions=[
+ "5.0.dev0",
+ "4.0",
+ "3.0",
+ "2.0",
+ "1.0",
+ ],
+ latest_version="4.0",
summary="This is my summary",
description="This is my description",
author="Jane Author",
| Fixup indexing query
Our current indexing query, https://github.com/pypa/warehouse/blob/f6deb957b37a5c2f742ab9e97019b3828671a6d3/warehouse/search/tasks.py#L29-L54
Is kind of abhorrent in performance as it pulls far to many rows (one for each project/version/classifier) and on top of that makes 2 queries to pull version history.
I have some SQL:
```sql
SELECT
releases.description AS releases_description,
releases.name AS releases_name,
releases.version AS releases_version,
(select array_agg(version) from releases as r where r.name = releases.name) as all_versions,
releases.author AS releases_author,
releases.author_email AS releases_author_email,
releases.maintainer AS releases_maintainer,
releases.maintainer_email AS releases_maintainer_email,
releases.home_page AS releases_home_page,
releases.summary AS releases_summary,
releases.keywords AS releases_keywords,
releases.platform AS releases_platform,
releases.download_url AS releases_download_url,
releases.created AS releases_created,
(select array_agg(id) from release_classifiers join trove_classifiers on trove_classifiers.id = release_classifiers.trove_id where releases.name = release_classifiers.name and releases.version = release_classifiers.version) as trove_classifiers_1_id,
(select array_agg(trove_classifiers.classifier) from release_classifiers join trove_classifiers on trove_classifiers.id = release_classifiers.trove_id where releases.name = release_classifiers.name and releases.version = release_classifiers.version) as trove_classifiers_1_id,
normalize_pep426_name(packages_1.name) AS normalize_pep426_name_1,
packages_1.name AS packages_1_name
FROM (
SELECT DISTINCT ON (releases.name) releases.name AS name, releases.version AS version FROM releases ORDER BY releases.name, pep440_is_prerelease(releases.version) NULLS LAST, releases._pypi_ordering DESC) AS release_list, releases
LEFT OUTER JOIN packages AS packages_1 ON packages_1.name = releases.name
WHERE releases.name = release_list.name AND releases.version = release_list.version
ORDER BY releases.name
```
Thanks to the help of @eeeebbbbrrrr that pulls what we need in a single row!
Can anyone help me convert the above query into SQLAlchemy so we can continue to use the windowed_query helper https://github.com/pypa/warehouse/blob/f6deb957b37a5c2f742ab9e97019b3828671a6d3/warehouse/search/tasks.py#L56
| ```shell
warehouse=> SELECT
warehouse-> -- releases.description AS releases_description,
warehouse-> releases.name AS releases_name,
warehouse-> releases.version AS releases_version,
warehouse-> (select array_agg(version) from releases as r where r.name = releases.name) as all_versions,
warehouse-> releases.author AS releases_author,
warehouse-> releases.author_email AS releases_author_email,
warehouse-> releases.maintainer AS releases_maintainer,
warehouse-> releases.maintainer_email AS releases_maintainer_email,
warehouse-> releases.home_page AS releases_home_page,
warehouse-> releases.summary AS releases_summary,
warehouse-> releases.keywords AS releases_keywords,
warehouse-> releases.platform AS releases_platform,
warehouse-> releases.download_url AS releases_download_url,
warehouse-> releases.created AS releases_created,
warehouse-> (select array_agg(id) from release_classifiers join trove_classifiers on trove_classifiers.id = release_classifiers.trove_id where releases.name = release_classifiers.name and releases.version = release_classifiers.version) as trove_classifiers_1_id,
warehouse-> (select array_agg(trove_classifiers.classifier) from release_classifiers join trove_classifiers on trove_classifiers.id = release_classifiers.trove_id where releases.name = release_classifiers.name and releases.version = release_classifiers.version) as trove_classifiers_1_id,
warehouse-> normalize_pep426_name(packages_1.name) AS normalize_pep426_name_1,
warehouse-> packages_1.name AS packages_1_name
warehouse-> FROM (
warehouse(> SELECT DISTINCT ON (releases.name) releases.name AS name, releases.version AS version FROM releases ORDER BY releases.name, pep440_is_prerelease(releases.version) NULLS LAST, releases._pypi_ordering DESC) AS release_list, releases
warehouse-> LEFT OUTER JOIN packages AS packages_1 ON packages_1.name = releases.name
warehouse-> WHERE releases.name = release_list.name AND releases.version = release_list.version AND releases.name = 'pip'
warehouse-> ORDER BY releases.name;
-[ RECORD 1 ]-------------+------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
releases_name | pip
releases_version | 10.0.0
all_versions | {9.0.2,9.0.1,0.8.1,0.6.1,0.6.3,0.5,1.2.1,6.0.7,1.3,8.0.2,6.1.0,7.0.3,0.7.2,7.0.1,6.0.3,0.7.1,0.7,1.4,6.0.8,0.8.3,6.0.4,0.5.1,1.0.2,1.5.1,1.5.6,8.0.1,0.6.2,0.8,1.5.2,1.5,6.0.2,1.3.1,8.1.1,6.0.1,8.0.0,8.0.3,0.8.2,7.1.2,6.1.1,0.4,7.1.1,6.0,0.2,0.3.1,7.1.0,1.2,1.4.1,1.1,0.2.1,1.5.4,0.3,8.1.0,0.6,1.5.3,9.0.0,8.1.2,6.0.6,1.5.5,6.0.5,1.0.1,1.0,7.0.2,7.0.0,9.0.3,10.0.0b1,10.0.0b2,10.0.0}
releases_author | The pip developers
releases_author_email | [email protected]
releases_maintainer |
releases_maintainer_email |
releases_home_page | https://pip.pypa.io/
releases_summary | The PyPA recommended tool for installing Python packages.
releases_keywords | easy_install distutils setuptools egg virtualenv
releases_platform |
releases_download_url |
releases_created | 2018-04-14 11:38:05.342706
trove_classifiers_1_id | {611,607,587,566,554,553,533,532,527,30,5,69,214,408}
trove_classifiers_1_id | {"Programming Language :: Python :: 3.6","Programming Language :: Python :: 3.5","Programming Language :: Python :: 3.4","Programming Language :: Python :: 3.3","Programming Language :: Python :: Implementation :: PyPy","Programming Language :: Python :: Implementation :: CPython","Programming Language :: Python :: 3","Programming Language :: Python :: 2.7","Programming Language :: Python :: 2","Intended Audience :: Developers","Development Status :: 5 - Production/Stable","License :: OSI Approved :: MIT License","Programming Language :: Python","Topic :: Software Development :: Build Tools"}
normalize_pep426_name_1 | pip
packages_1_name | pip
```
Query pulls everything we need in a single row! Performs well! (Description not queried for clarity)
I don't think the `all_versions` column requires ordering as to my knowledge it isn't exposed to users, just used for search.
But if it does, we can just reorder it when constructing the document.
I'm taking a look at this | 2018-04-15T00:31:39Z | [] | [] |
pypi/warehouse | 3,771 | pypi__warehouse-3771 | [
"1300"
] | e26ec6cb893741a0df7bf3010bf1308638b4f2b6 | diff --git a/warehouse/admin/routes.py b/warehouse/admin/routes.py
--- a/warehouse/admin/routes.py
+++ b/warehouse/admin/routes.py
@@ -102,6 +102,11 @@ def includeme(config):
'/admin/classifiers/add/',
domain=warehouse,
)
+ config.add_route(
+ 'admin.classifiers.deprecate',
+ '/admin/classifiers/deprecate/',
+ domain=warehouse,
+ )
# Blacklist related Admin pages
config.add_route(
diff --git a/warehouse/admin/views/classifiers.py b/warehouse/admin/views/classifiers.py
--- a/warehouse/admin/views/classifiers.py
+++ b/warehouse/admin/views/classifiers.py
@@ -25,7 +25,6 @@
def get_classifiers(request):
classifiers = (
request.db.query(Classifier)
- .filter(Classifier.l5 == 0) # Can be a parent
.order_by(Classifier.classifier)
.all()
)
@@ -97,3 +96,28 @@ def add_child_classifier(self):
)
return HTTPSeeOther(self.request.route_path('admin.classifiers'))
+
+
+@view_config(
+ route_name='admin.classifiers.deprecate',
+ permission='admin',
+ request_method='POST',
+ uses_session=True,
+ require_methods=False,
+ require_csrf=True,
+)
+def deprecate_classifier(request):
+ classifier = (
+ request.db
+ .query(Classifier)
+ .get(request.params.get('classifier_id'))
+ )
+
+ classifier.deprecated = True
+
+ request.session.flash(
+ f'Successfully deprecated classifier {classifier.classifier!r}',
+ queue='success',
+ )
+
+ return HTTPSeeOther(request.route_path('admin.classifiers'))
diff --git a/warehouse/classifiers/models.py b/warehouse/classifiers/models.py
--- a/warehouse/classifiers/models.py
+++ b/warehouse/classifiers/models.py
@@ -10,7 +10,7 @@
# See the License for the specific language governing permissions and
# limitations under the License.
-from sqlalchemy import Column, Index, Integer, Text
+from sqlalchemy import Boolean, Column, Index, Integer, Text, sql
from warehouse import db
from warehouse.utils.attrs import make_repr
@@ -28,6 +28,7 @@ class Classifier(db.ModelBase):
id = Column(Integer, primary_key=True, nullable=False)
classifier = Column(Text, unique=True)
+ deprecated = Column(Boolean, nullable=False, server_default=sql.false())
l2 = Column(Integer)
l3 = Column(Integer)
l4 = Column(Integer)
diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -696,6 +696,32 @@ def _is_duplicate_file(db_session, filename, hashes):
return None
+def _no_deprecated_classifiers(request):
+ deprecated_classifiers = {
+ classifier.classifier
+ for classifier in (
+ request.db.query(Classifier.classifier)
+ .filter(Classifier.deprecated.is_(True))
+ .all()
+ )
+ }
+
+ def validate_no_deprecated_classifiers(form, field):
+ invalid_classifiers = set(field.data or []) & deprecated_classifiers
+ if invalid_classifiers:
+ first_invalid_classifier = sorted(invalid_classifiers)[0]
+ host = request.registry.settings.get("warehouse.domain")
+ classifiers_url = request.route_url('classifiers', _host=host)
+
+ raise wtforms.validators.ValidationError(
+ f'Classifier {first_invalid_classifier!r} has been '
+ f'deprecated, see {classifiers_url} for a list of valid '
+ 'classifiers.'
+ )
+
+ return validate_no_deprecated_classifiers
+
+
@view_config(
route_name="forklift.legacy.file_upload",
uses_session=True,
@@ -757,6 +783,9 @@ def file_upload(request):
# Validate and process the incoming metadata.
form = MetadataForm(request.POST)
+ # Add a validator for deprecated classifiers
+ form.classifiers.validators.append(_no_deprecated_classifiers(request))
+
form.classifiers.choices = [
(c.classifier, c.classifier) for c in all_classifiers
]
diff --git a/warehouse/legacy/api/pypi.py b/warehouse/legacy/api/pypi.py
--- a/warehouse/legacy/api/pypi.py
+++ b/warehouse/legacy/api/pypi.py
@@ -81,8 +81,9 @@ def forbidden_legacy(exc, request):
def list_classifiers(request):
classifiers = (
request.db.query(Classifier.classifier)
- .order_by(Classifier.classifier)
- .all()
+ .filter(Classifier.deprecated.is_(False))
+ .order_by(Classifier.classifier)
+ .all()
)
return Response(
diff --git a/warehouse/migrations/versions/e0ca60b6a30b_add_deprecated_column_to_classifiers.py b/warehouse/migrations/versions/e0ca60b6a30b_add_deprecated_column_to_classifiers.py
new file mode 100644
--- /dev/null
+++ b/warehouse/migrations/versions/e0ca60b6a30b_add_deprecated_column_to_classifiers.py
@@ -0,0 +1,41 @@
+# Licensed under the Apache License, Version 2.0 (the "License");
+# you may not use this file except in compliance with the License.
+# You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing, software
+# distributed under the License is distributed on an "AS IS" BASIS,
+# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+# See the License for the specific language governing permissions and
+# limitations under the License.
+"""
+Add 'deprecated' column to classifiers
+
+Revision ID: e0ca60b6a30b
+Revises: 6714f3f04f0f
+Create Date: 2018-04-18 23:24:13.009357
+"""
+
+from alembic import op
+import sqlalchemy as sa
+
+
+revision = "e0ca60b6a30b"
+down_revision = "6714f3f04f0f"
+
+
+def upgrade():
+ op.add_column(
+ "trove_classifiers",
+ sa.Column(
+ "deprecated",
+ sa.Boolean(),
+ server_default=sa.text("false"),
+ nullable=False,
+ ),
+ )
+
+
+def downgrade():
+ op.drop_column("trove_classifiers", "deprecated")
diff --git a/warehouse/routes.py b/warehouse/routes.py
--- a/warehouse/routes.py
+++ b/warehouse/routes.py
@@ -83,6 +83,9 @@ def includeme(config):
domain=warehouse,
)
+ # Classifier Routes
+ config.add_route("classifiers", "/classifiers/", domain=warehouse)
+
# Search Routes
config.add_route("search", "/search/", domain=warehouse)
diff --git a/warehouse/views.py b/warehouse/views.py
--- a/warehouse/views.py
+++ b/warehouse/views.py
@@ -235,6 +235,23 @@ def index(request):
}
+@view_config(
+ route_name="classifiers",
+ renderer="pages/classifiers.html",
+)
+def classifiers(request):
+ classifiers = (
+ request.db.query(Classifier.classifier)
+ .filter(Classifier.deprecated.is_(False))
+ .order_by(Classifier.classifier)
+ .all()
+ )
+
+ return {
+ 'classifiers': classifiers
+ }
+
+
@view_config(
route_name="search",
renderer="search/results.html",
@@ -309,6 +326,7 @@ def search(request):
classifiers_q = (
request.db.query(Classifier)
.with_entities(Classifier.classifier)
+ .filter(Classifier.deprecated.is_(False))
.filter(
exists([release_classifiers.c.trove_id])
.where(release_classifiers.c.trove_id == Classifier.id)
| diff --git a/tests/unit/admin/test_routes.py b/tests/unit/admin/test_routes.py
--- a/tests/unit/admin/test_routes.py
+++ b/tests/unit/admin/test_routes.py
@@ -101,6 +101,11 @@ def test_includeme():
'/admin/classifiers/add/',
domain=warehouse,
),
+ pretend.call(
+ 'admin.classifiers.deprecate',
+ '/admin/classifiers/deprecate/',
+ domain=warehouse,
+ ),
pretend.call(
"admin.blacklist.list",
"/admin/blacklist/",
diff --git a/tests/unit/admin/views/test_classifiers.py b/tests/unit/admin/views/test_classifiers.py
--- a/tests/unit/admin/views/test_classifiers.py
+++ b/tests/unit/admin/views/test_classifiers.py
@@ -22,9 +22,8 @@
class TestGetClassifiers:
def test_get_classifiers(self, db_request):
- classifier_a = ClassifierFactory(l5=0, classifier='I am first')
- classifier_b = ClassifierFactory(l5=0, classifier='I am last')
- ClassifierFactory(l5=1) # Ignored because it has a nonzero L5
+ classifier_a = ClassifierFactory(classifier='I am first')
+ classifier_b = ClassifierFactory(classifier='I am last')
assert views.get_classifiers(db_request) == {
'classifiers': [classifier_a, classifier_b],
@@ -89,3 +88,18 @@ def test_add_parent_classifier(self, db_request):
assert new.l3 == 0
assert new.l4 == 0
assert new.l5 == 0
+
+
+class TestDeprecateClassifier:
+
+ def test_deprecate_classifier(self, db_request):
+ classifier = ClassifierFactory(deprecated=False)
+
+ db_request.params = {'classifier_id': classifier.id}
+ db_request.session.flash = pretend.call_recorder(lambda *a, **kw: None)
+ db_request.route_path = lambda *a: '/the/path'
+
+ views.deprecate_classifier(db_request)
+ db_request.db.flush()
+
+ assert classifier.deprecated
diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -39,6 +39,7 @@
from warehouse.admin.flags import AdminFlag
from ...common.db.accounts import UserFactory, EmailFactory
+from ...common.db.classifiers import ClassifierFactory
from ...common.db.packaging import (
ProjectFactory, ReleaseFactory, FileFactory, RoleFactory,
)
@@ -326,6 +327,31 @@ def test_validate_description_content_type_invalid(self, data):
with pytest.raises(ValidationError):
legacy._validate_description_content_type(form, field)
+ def test_validate_no_deprecated_classifiers_valid(self, db_request):
+ valid_classifier = ClassifierFactory(deprecated=False)
+ validator = legacy._no_deprecated_classifiers(db_request)
+
+ form = pretend.stub()
+ field = pretend.stub(data=[valid_classifier.classifier])
+
+ validator(form, field)
+
+ def test_validate_no_deprecated_classifiers_invalid(self, db_request):
+ deprecated_classifier = ClassifierFactory(
+ classifier='AA: BB', deprecated=True,
+ )
+ validator = legacy._no_deprecated_classifiers(db_request)
+ db_request.registry = pretend.stub(
+ settings={'warehouse.domain': 'host'}
+ )
+ db_request.route_url = pretend.call_recorder(lambda *a, **kw: '/url')
+
+ form = pretend.stub()
+ field = pretend.stub(data=[deprecated_classifier.classifier])
+
+ with pytest.raises(ValidationError):
+ validator(form, field)
+
def test_construct_dependencies():
types = {
@@ -1522,6 +1548,48 @@ def test_upload_fails_with_invalid_classifier(self, pyramid_config,
"for this field"
)
+ def test_upload_fails_with_deprecated_classifier(
+ self, pyramid_config, db_request):
+ pyramid_config.testing_securitypolicy(userid=1)
+
+ user = UserFactory.create()
+ EmailFactory.create(user=user)
+ project = ProjectFactory.create()
+ release = ReleaseFactory.create(project=project, version="1.0")
+ RoleFactory.create(user=user, project=project)
+ classifier = ClassifierFactory(classifier='AA :: BB', deprecated=True)
+
+ filename = "{}-{}.tar.gz".format(project.name, release.version)
+
+ db_request.POST = MultiDict({
+ "metadata_version": "1.2",
+ "name": project.name,
+ "version": release.version,
+ "filetype": "sdist",
+ "md5_digest": "335c476dc930b959dda9ec82bd65ef19",
+ "content": pretend.stub(
+ filename=filename,
+ file=io.BytesIO(b"A fake file."),
+ type="application/tar",
+ ),
+ })
+ db_request.POST.extend([
+ ("classifiers", classifier.classifier),
+ ])
+ db_request.route_url = pretend.call_recorder(lambda *a, **kw: '/url')
+
+ with pytest.raises(HTTPBadRequest) as excinfo:
+ legacy.file_upload(db_request)
+
+ resp = excinfo.value
+
+ assert resp.status_code == 400
+ assert resp.status == (
+ "400 Invalid value for classifiers. "
+ "Error: Classifier 'AA :: BB' has been deprecated, see /url "
+ "for a list of valid classifiers."
+ )
+
@pytest.mark.parametrize(
"digests",
[
diff --git a/tests/unit/test_routes.py b/tests/unit/test_routes.py
--- a/tests/unit/test_routes.py
+++ b/tests/unit/test_routes.py
@@ -114,6 +114,7 @@ def add_policy(name, filename):
traverse="/{username}",
domain=warehouse,
),
+ pretend.call("classifiers", "/classifiers/", domain=warehouse),
pretend.call("search", "/search/", domain=warehouse),
pretend.call(
"accounts.profile",
diff --git a/tests/unit/test_views.py b/tests/unit/test_views.py
--- a/tests/unit/test_views.py
+++ b/tests/unit/test_views.py
@@ -23,9 +23,9 @@
from warehouse import views
from warehouse.views import (
- SEARCH_BOOSTS, SEARCH_FIELDS, current_user_indicator, forbidden, health,
- httpexception_view, index, robotstxt, opensearchxml, search, force_status,
- flash_messages, forbidden_include
+ SEARCH_BOOSTS, SEARCH_FIELDS, classifiers, current_user_indicator,
+ forbidden, health, httpexception_view, index, robotstxt, opensearchxml,
+ search, force_status, flash_messages, forbidden_include
)
from ..common.db.accounts import UserFactory
@@ -545,6 +545,15 @@ def test_raises_400_with_pagenum_type_str(self, monkeypatch, db_request):
assert page_cls.calls == []
+def test_classifiers(db_request):
+ classifier_a = ClassifierFactory(classifier='I am first')
+ classifier_b = ClassifierFactory(classifier='I am last')
+
+ assert classifiers(db_request) == {
+ 'classifiers': [(classifier_a.classifier,), (classifier_b.classifier,)]
+ }
+
+
def test_health():
request = pretend.stub(
db=pretend.stub(
| Add page with list of trove classifiers
For package authors, we will need to add a list of all trove classifiers. I am not sure where this is best placed - maybe in the footer?
ping @dstufft for an opinion on this.
| Is this really a page that belongs on the PyPI site?
[PEP 301](https://www.python.org/dev/peps/pep-0301/#distutils-trove-classification) calls them _**distutils** trove classifiers_. Maybe a better place to give more information about those to the package authors would be the [Python Packaging User Guide](https://packaging.python.org/)?
I think a link somewhere close to the classifiers shown in the project details page would be useful.
Yes, sure. I am not opposed to moving it out of the site and into the docs. I do think we need to continue to link directly to it though, as this is something that is currently done on PyPI (so users may be accustomed to finding the list via this link).
It's valuable to have the list in a convenient plain form, not embedded in HTML docs, for tools to fetch. Flit does this, for instance. The list changes often enough (e.g. adding new frameworks) that I don't want to bundle a static copy.
At present it seems you can get this from the legacy API at https://pypi.org/pypi?%3Aaction=list_classifiers - can we rely on that URL working for the foreseeable future?
Yes that will continue to work, although ideally for automated consumption we come up with some newer, better JSON based API.
Great, thanks. There's no pressure from my side for a JSON version of this unless someone makes a classifier with a newline in.
I think @timofurrer's question does raise an interesting UX question: would a file in the specifications section of the PyPUG and/or some other PyPA repo that anyone can submit a PR to be a better primary data source for this information than the PyPI database?
Then PyPI would just be a consumer of that file (presenting it via the web URL), rather than the source of the official list. | 2018-04-19T11:42:12Z | [] | [] |
pypi/warehouse | 3,778 | pypi__warehouse-3778 | [
"3734"
] | f7d6a6331a22adaae34da9af5c714e1a74ecff4d | diff --git a/warehouse/legacy/api/xmlrpc/views.py b/warehouse/legacy/api/xmlrpc/views.py
--- a/warehouse/legacy/api/xmlrpc/views.py
+++ b/warehouse/legacy/api/xmlrpc/views.py
@@ -13,10 +13,12 @@
import collections.abc
import datetime
import functools
+import xmlrpc.client
import xmlrpc.server
from elasticsearch_dsl import Q
from packaging.utils import canonicalize_name
+from pyramid.request import Request
from pyramid.view import view_config
from pyramid_rpc.xmlrpc import (
exception_view as _exception_view, xmlrpc_method as _xmlrpc_method
@@ -31,6 +33,9 @@
)
+_MAX_MULTICALLS = 20
+
+
def xmlrpc_method(**kwargs):
"""
Support multiple endpoints serving the same views by chaining calls to
@@ -441,3 +446,49 @@ def browse(request, classifiers):
)
return [(r.name, r.version) for r in releases]
+
+
+def measure_response_content_length(metric_name):
+
+ def _callback(request, response):
+ request.registry.datadog.histogram(
+ metric_name, response.content_length
+ )
+
+ return _callback
+
+
+@xmlrpc_method(method='system.multicall')
+def multicall(request, args):
+ if any(arg.get('methodName') == 'system.multicall' for arg in args):
+ raise XMLRPCWrappedError(
+ ValueError('Cannot use system.multicall inside a multicall')
+ )
+
+ if not all(arg.get('methodName') for arg in args):
+ raise XMLRPCWrappedError(ValueError('Method name not provided'))
+
+ if len(args) > _MAX_MULTICALLS:
+ raise XMLRPCWrappedError(
+ ValueError(f'Multicall limit is {_MAX_MULTICALLS} calls')
+ )
+
+ responses = []
+ for arg in args:
+ name = arg.get('methodName')
+ subreq = Request.blank('/RPC2', headers={'Content-Type': 'text/xml'})
+ subreq.method = 'POST'
+ subreq.body = xmlrpc.client.dumps(
+ tuple(arg.get('params')),
+ methodname=name,
+ ).encode()
+ response = request.invoke_subrequest(subreq, use_tweens=True)
+ responses.append(xmlrpc.client.loads(response.body))
+
+ request.add_response_callback(
+ measure_response_content_length(
+ 'warehouse.xmlrpc.system.multicall.content_length'
+ )
+ )
+
+ return responses
| diff --git a/tests/unit/legacy/api/xmlrpc/test_xmlrpc.py b/tests/unit/legacy/api/xmlrpc/test_xmlrpc.py
--- a/tests/unit/legacy/api/xmlrpc/test_xmlrpc.py
+++ b/tests/unit/legacy/api/xmlrpc/test_xmlrpc.py
@@ -792,3 +792,112 @@ def test_browse(db_request):
"Programming Language :: Python",
],
)) == {(expected_release.name, expected_release.version)}
+
+
+class TestMulticall:
+
+ def test_multicall(self, monkeypatch):
+ dumped = pretend.stub(encode=lambda: None)
+ dumps = pretend.call_recorder(lambda *a, **kw: dumped)
+ monkeypatch.setattr(xmlrpc.xmlrpc.client, 'dumps', dumps)
+
+ loaded = pretend.stub()
+ loads = pretend.call_recorder(lambda *a, **kw: loaded)
+ monkeypatch.setattr(xmlrpc.xmlrpc.client, 'loads', loads)
+
+ subreq = pretend.stub()
+ blank = pretend.call_recorder(lambda *a, **kw: subreq)
+ monkeypatch.setattr(xmlrpc.Request, 'blank', blank)
+
+ body = pretend.stub()
+ response = pretend.stub(body=body)
+
+ request = pretend.stub(
+ invoke_subrequest=pretend.call_recorder(lambda *a, **kw: response),
+ add_response_callback=pretend.call_recorder(
+ lambda *a, **kw: response),
+ )
+
+ callback = pretend.stub()
+ monkeypatch.setattr(
+ xmlrpc,
+ 'measure_response_content_length',
+ pretend.call_recorder(lambda metric_name: callback)
+ )
+
+ args = [
+ {'methodName': 'search', 'params': [{'name': 'foo'}]},
+ {'methodName': 'browse', 'params': [{'classifiers': ['bar']}]},
+ ]
+
+ responses = xmlrpc.multicall(request, args)
+
+ assert responses == [loaded, loaded]
+ assert blank.calls == [
+ pretend.call('/RPC2', headers={'Content-Type': 'text/xml'}),
+ pretend.call('/RPC2', headers={'Content-Type': 'text/xml'}),
+ ]
+ assert request.invoke_subrequest.calls == [
+ pretend.call(subreq, use_tweens=True),
+ pretend.call(subreq, use_tweens=True),
+ ]
+ assert request.add_response_callback.calls == [
+ pretend.call(callback),
+ ]
+ assert dumps.calls == [
+ pretend.call(({'name': 'foo'},), methodname='search'),
+ pretend.call(({'classifiers': ['bar']},), methodname='browse'),
+ ]
+ assert loads.calls == [pretend.call(body), pretend.call(body)]
+
+ def test_recursive_multicall(self):
+ request = pretend.stub()
+ args = [
+ {'methodName': 'system.multicall', 'params': []},
+ ]
+ with pytest.raises(xmlrpc.XMLRPCWrappedError) as exc:
+ xmlrpc.multicall(request, args)
+
+ assert exc.value.faultString == (
+ 'ValueError: Cannot use system.multicall inside a multicall'
+ )
+
+ def test_missing_multicall_method(self):
+ request = pretend.stub()
+ args = [{}]
+ with pytest.raises(xmlrpc.XMLRPCWrappedError) as exc:
+ xmlrpc.multicall(request, args)
+
+ assert exc.value.faultString == (
+ 'ValueError: Method name not provided'
+ )
+
+ def test_too_many_multicalls_method(self):
+ request = pretend.stub()
+ args = [{'methodName': 'nah'}] * 21
+
+ with pytest.raises(xmlrpc.XMLRPCWrappedError) as exc:
+ xmlrpc.multicall(request, args)
+
+ assert exc.value.faultString == (
+ 'ValueError: Multicall limit is 20 calls'
+ )
+
+ def test_measure_response_content_length(self):
+ metric_name = 'some_metric_name'
+ callback = xmlrpc.measure_response_content_length(metric_name)
+
+ request = pretend.stub(
+ registry=pretend.stub(
+ datadog=pretend.stub(
+ histogram=pretend.call_recorder(lambda *a: None)
+ )
+ )
+ )
+ response = pretend.stub(content_length=pretend.stub())
+
+ callback(request, response)
+
+ assert request.registry.datadog.histogram.calls == [
+ pretend.call(metric_name, response.content_length),
+ ]
| XML-RPC doesn't support MultiCall
New API differ from the old API (`pypi.python.org/pypi/`):
* ~`search` method work different e.g. `search({'keywords': "orange3 add-on"})` returns empty list because of space~
* `MultiCall` is not supported.
I know that this API is deprecated but would ask if there is an option to make functionalities work same to support old versions of the softwares.
| Search issue is likely #3717
MultiCall must not be supported by our RPC implementation, https://github.com/Pylons/pyramid_rpc
@PrimozGodec if you point to https://legacy.pypi.org does that address your needs for the time being, and eventually do you want/expect the new API to support some of the same functionality?
FTR, something like this (untested) in warehouse/legacy/api/xmlrpc/views.py is one way to go to support this:
```
@xmlrpc_method(method='system.multicall')
def multicall(request):
args = request.rpc_args
responses = []
for arg in args:
name = arg.get('methodName')
params = arg.get('params')
if name == 'system.multicall':
raise Exception
if not name:
raise Exception
subreq = Request.blank('/RPC2', base_url=request.application_url)
subreq.method = 'POST'
subreq.body = xmlrpclib.dumps(arg)
response = request.invoke_subrequest(subreq, use_tweens=True)
responses.append(xmlrpclib.loads(response.body))
return responses
``` | 2018-04-20T20:18:28Z | [] | [] |
pypi/warehouse | 3,800 | pypi__warehouse-3800 | [
"3799"
] | 5e5d28bf6a19d7d47527583c22cf9317e5d298ab | diff --git a/warehouse/legacy/api/pypi.py b/warehouse/legacy/api/pypi.py
--- a/warehouse/legacy/api/pypi.py
+++ b/warehouse/legacy/api/pypi.py
@@ -10,7 +10,7 @@
# See the License for the specific language governing permissions and
# limitations under the License.
-from pyramid.httpexceptions import HTTPGone
+from pyramid.httpexceptions import HTTPGone, HTTPMovedPermanently, HTTPNotFound
from pyramid.response import Response
from pyramid.view import forbidden_view_config, view_config
@@ -90,3 +90,31 @@ def list_classifiers(request):
text='\n'.join(c[0] for c in classifiers),
content_type='text/plain; charset=utf-8'
)
+
+
+@view_config(route_name='legacy.api.pypi.search')
+def search(request):
+ return HTTPMovedPermanently(
+ request.route_path(
+ 'search', _query={'q': request.params.get('term')}
+ )
+ )
+
+
+@view_config(route_name='legacy.api.pypi.browse')
+def browse(request):
+ classifier_id = request.params.get('c')
+
+ if not classifier_id:
+ raise HTTPNotFound
+
+ classifier = request.db.query(Classifier).get(classifier_id)
+
+ if not classifier:
+ raise HTTPNotFound
+
+ return HTTPMovedPermanently(
+ request.route_path(
+ 'search', _query={'c': classifier.classifier}
+ )
+ )
diff --git a/warehouse/routes.py b/warehouse/routes.py
--- a/warehouse/routes.py
+++ b/warehouse/routes.py
@@ -279,6 +279,16 @@ def includeme(config):
"list_classifiers",
domain=warehouse,
)
+ config.add_pypi_action_route(
+ 'legacy.api.pypi.search',
+ 'search',
+ domain=warehouse,
+ )
+ config.add_pypi_action_route(
+ 'legacy.api.pypi.browse',
+ 'browse',
+ domain=warehouse,
+ )
# Legacy XMLRPC
config.add_xmlrpc_endpoint(
| diff --git a/tests/unit/legacy/api/test_pypi.py b/tests/unit/legacy/api/test_pypi.py
--- a/tests/unit/legacy/api/test_pypi.py
+++ b/tests/unit/legacy/api/test_pypi.py
@@ -13,7 +13,9 @@
import pretend
import pytest
-from pyramid.httpexceptions import HTTPBadRequest
+from pyramid.httpexceptions import (
+ HTTPBadRequest, HTTPMovedPermanently, HTTPNotFound,
+)
from warehouse.legacy.api import pypi
@@ -80,3 +82,52 @@ def test_list_classifiers(db_request):
assert resp.status_code == 200
assert resp.text == "fiz :: buz\nfoo :: bar\nfoo :: baz"
+
+
+def test_search():
+ term = pretend.stub()
+ request = pretend.stub(
+ params={'term': term},
+ route_path=pretend.call_recorder(lambda *a, **kw: '/the/path'),
+ )
+
+ result = pypi.search(request)
+
+ assert isinstance(result, HTTPMovedPermanently)
+ assert result.headers['Location'] == '/the/path'
+ assert result.status_code == 301
+ assert request.route_path.calls == [
+ pretend.call('search', _query={'q': term}),
+ ]
+
+
+class TestBrowse:
+
+ def test_browse(self, db_request):
+ classifier = ClassifierFactory.create(classifier="foo :: bar")
+
+ db_request.params = {'c': str(classifier.id)}
+ db_request.route_path = pretend.call_recorder(
+ lambda *a, **kw: '/the/path'
+ )
+
+ result = pypi.browse(db_request)
+
+ assert isinstance(result, HTTPMovedPermanently)
+ assert result.headers['Location'] == '/the/path'
+ assert result.status_code == 301
+ assert db_request.route_path.calls == [
+ pretend.call('search', _query={'c': classifier.classifier}),
+ ]
+
+ def test_browse_no_id(self):
+ request = pretend.stub(params={})
+
+ with pytest.raises(HTTPNotFound):
+ pypi.browse(request)
+
+ def test_browse_bad_id(self, db_request):
+ db_request.params = {'c': '99999'}
+
+ with pytest.raises(HTTPNotFound):
+ pypi.browse(db_request)
diff --git a/tests/unit/test_routes.py b/tests/unit/test_routes.py
--- a/tests/unit/test_routes.py
+++ b/tests/unit/test_routes.py
@@ -322,6 +322,16 @@ def add_policy(name, filename):
"list_classifiers",
domain=warehouse,
),
+ pretend.call(
+ 'legacy.api.pypi.search',
+ 'search',
+ domain=warehouse,
+ ),
+ pretend.call(
+ 'legacy.api.pypi.browse',
+ 'browse',
+ domain=warehouse,
+ ),
]
assert config.add_pypi_action_redirect.calls == [
| Redirect legacy classifier filter URLs
Some projects' documentation ([example](https://github.com/sphinx-doc/sphinx/pull/4891)) points to legacy PyPI URLs for classifier-based searches like
https://pypi.python.org/pypi/?:action=browse&c=599
Could we have those redirect?
| @dstufft @di thoughts here?
I'm neutral here. If there is a trivial way of supporting a URL rewrite we should consider it.
As far as I know, archival services do not generally respect query parameters so while we would be breaking links... I'm not sure how damaging this is to the web overall.
It wouldn't be hard to support I don't think. You're just adding another legacy API in ``warehouse/legacy/api/pypi.py`` and looking up the classifier by ID, and redirecting to the search page for that classifier.
I think this is totally doable, see what we do for `list_classifiers` here: https://github.com/pypa/warehouse/blob/d8269247e43123feeeb7632d89f4698b58f1cb8a/warehouse/legacy/api/pypi.py#L80-L92
Note that regular search links (without classifiers) should probably be supported as well, e.g:
https://legacy.pypi.org/pypi?%3Aaction=search&term=foobar&submit=search
should redirect to:
https://pypi.org/search/?q=foobar | 2018-04-24T20:14:58Z | [] | [] |
pypi/warehouse | 3,820 | pypi__warehouse-3820 | [
"3798"
] | 047ec675e66431f9178048d1767958fb3af52aa5 | diff --git a/warehouse/legacy/api/json.py b/warehouse/legacy/api/json.py
--- a/warehouse/legacy/api/json.py
+++ b/warehouse/legacy/api/json.py
@@ -154,6 +154,7 @@ def json_release(release, request):
"name": project.name,
"version": release.version,
"summary": release.summary,
+ "description_content_type": release.description_content_type,
"description": release.description,
"keywords": release.keywords,
"license": release.license,
| diff --git a/tests/unit/legacy/api/test_json.py b/tests/unit/legacy/api/test_json.py
--- a/tests/unit/legacy/api/test_json.py
+++ b/tests/unit/legacy/api/test_json.py
@@ -141,10 +141,19 @@ def test_normalizing_redirects(self, db_request):
def test_detail_renders(self, pyramid_config, db_request):
project = ProjectFactory.create(has_docs=True)
+ description_content_type = "text/x-rst"
releases = [
ReleaseFactory.create(project=project, version=v)
- for v in ["1.0", "2.0", "3.0"]
+ for v in ["0.1", "1.0", "2.0"]
]
+ releases += [
+ ReleaseFactory.create(
+ project=project,
+ version="3.0",
+ description_content_type=description_content_type,
+ )
+ ]
+
files = [
FileFactory.create(
release=r,
@@ -153,7 +162,7 @@ def test_detail_renders(self, pyramid_config, db_request):
size=200,
has_signature=True,
)
- for r in releases[:-1]
+ for r in releases[1:]
]
user = UserFactory.create()
JournalEntryFactory.reset_sequence()
@@ -165,16 +174,17 @@ def test_detail_renders(self, pyramid_config, db_request):
url = "/the/fake/url/"
db_request.route_url = pretend.call_recorder(lambda *args, **kw: url)
- result = json.json_release(releases[1], db_request)
+ result = json.json_release(releases[3], db_request)
assert set(db_request.route_url.calls) == {
pretend.call("packaging.file", path=files[0].path),
pretend.call("packaging.file", path=files[1].path),
+ pretend.call("packaging.file", path=files[2].path),
pretend.call("packaging.project", name=project.name),
pretend.call(
"packaging.release",
name=project.name,
- version=releases[1].version,
+ version=releases[3].version,
),
pretend.call("legacy.docs", project=project.name),
}
@@ -188,6 +198,7 @@ def test_detail_renders(self, pyramid_config, db_request):
"author_email": None,
"bugtrack_url": None,
"classifiers": [],
+ "description_content_type": description_content_type,
"description": None,
"docs_url": "/the/fake/url/",
"download_url": None,
@@ -209,9 +220,10 @@ def test_detail_renders(self, pyramid_config, db_request):
"requires_dist": None,
"requires_python": None,
"summary": None,
- "version": "2.0",
+ "version": "3.0",
},
"releases": {
+ "0.1": [],
"1.0": [
{
"comment_text": None,
@@ -252,23 +264,153 @@ def test_detail_renders(self, pyramid_config, db_request):
"url": "/the/fake/url/",
},
],
- "3.0": [],
+ "3.0": [
+ {
+ "comment_text": None,
+ "downloads": -1,
+ "filename": files[2].filename,
+ "has_sig": True,
+ "md5_digest": files[2].md5_digest,
+ "digests": {
+ "md5": files[2].md5_digest,
+ "sha256": files[2].sha256_digest,
+ },
+ "packagetype": None,
+ "python_version": "source",
+ "size": 200,
+ "upload_time": files[2].upload_time.strftime(
+ "%Y-%m-%dT%H:%M:%S",
+ ),
+ "url": "/the/fake/url/",
+ },
+ ],
+ },
+ "urls": [
+ {
+ "comment_text": None,
+ "downloads": -1,
+ "filename": files[2].filename,
+ "has_sig": True,
+ "md5_digest": files[2].md5_digest,
+ "digests": {
+ "md5": files[2].md5_digest,
+ "sha256": files[2].sha256_digest,
+ },
+ "packagetype": None,
+ "python_version": "source",
+ "size": 200,
+ "upload_time": files[2].upload_time.strftime(
+ "%Y-%m-%dT%H:%M:%S",
+ ),
+ "url": "/the/fake/url/",
+ },
+ ],
+ "last_serial": je.id,
+ }
+
+ def test_minimal_renders(self, pyramid_config, db_request):
+ project = ProjectFactory.create(has_docs=False)
+ release = ReleaseFactory.create(project=project, version="0.1")
+ file = FileFactory.create(
+ release=release,
+ filename="{}-{}.tar.gz".format(project.name, release.version),
+ python_version="source",
+ size=200,
+ has_signature=True,
+ )
+
+ user = UserFactory.create()
+ JournalEntryFactory.reset_sequence()
+ je = JournalEntryFactory.create(
+ name=project.name,
+ submitted_by=user,
+ )
+
+ url = "/the/fake/url/"
+ db_request.route_url = pretend.call_recorder(lambda *args, **kw: url)
+
+ result = json.json_release(release, db_request)
+
+ assert set(db_request.route_url.calls) == {
+ pretend.call("packaging.file", path=file.path),
+ pretend.call("packaging.project", name=project.name),
+ pretend.call(
+ "packaging.release",
+ name=project.name,
+ version=release.version,
+ ),
+ }
+
+ _assert_has_cors_headers(db_request.response.headers)
+ assert db_request.response.headers["X-PyPI-Last-Serial"] == str(je.id)
+
+ assert result == {
+ "info": {
+ "author": None,
+ "author_email": None,
+ "bugtrack_url": None,
+ "classifiers": [],
+ "description_content_type": None,
+ "description": None,
+ "docs_url": None,
+ "download_url": None,
+ "downloads": {
+ "last_day": -1,
+ "last_week": -1,
+ "last_month": -1,
+ },
+ "home_page": None,
+ "keywords": None,
+ "license": None,
+ "maintainer": None,
+ "maintainer_email": None,
+ "name": project.name,
+ "platform": None,
+ "project_url": "/the/fake/url/",
+ "package_url": "/the/fake/url/",
+ "release_url": "/the/fake/url/",
+ "requires_dist": None,
+ "requires_python": None,
+ "summary": None,
+ "version": "0.1",
+ },
+ "releases": {
+ "0.1": [
+ {
+ "comment_text": None,
+ "downloads": -1,
+ "filename": file.filename,
+ "has_sig": True,
+ "md5_digest": file.md5_digest,
+ "digests": {
+ "md5": file.md5_digest,
+ "sha256": file.sha256_digest,
+ },
+ "packagetype": None,
+ "python_version": "source",
+ "size": 200,
+ "upload_time": file.upload_time.strftime(
+ "%Y-%m-%dT%H:%M:%S",
+ ),
+ "url": "/the/fake/url/",
+ },
+ ],
},
"urls": [
{
"comment_text": None,
"downloads": -1,
- "filename": files[1].filename,
+ "filename": file.filename,
"has_sig": True,
- "md5_digest": files[1].md5_digest,
+ "md5_digest": file.md5_digest,
"digests": {
- "md5": files[1].md5_digest,
- "sha256": files[1].sha256_digest,
+ "md5": file.md5_digest,
+ "sha256": file.sha256_digest,
},
"packagetype": None,
"python_version": "source",
"size": 200,
- "upload_time": files[1].upload_time.strftime(
+ "upload_time": file.upload_time.strftime(
"%Y-%m-%dT%H:%M:%S",
),
"url": "/the/fake/url/",
| Expose long_description_content_type in JSON API
Feature request: Add 'long_description_content_type' to JSON API in 'info': section, alongside the existing 'description'
Now that PyPI supports Markdown and more than one kind of content type for long descriptions, it would be helpful to be able to see what the content type is with the JSON API. Otherwise it is more difficult to correctly interpret the 'description', since it isn't immediately obvious if it's reStructuredText or Markdown with which variant.
I'm willing to try this if I'm pointed in the right general direction :) If someone else can get it done trivially that's fine too.
| @nixjdm Sounds like a good idea. Here's where the `description` field is added: https://github.com/pypa/warehouse/blob/338d6a102939c6452c0b6825924151d1b8b09301/warehouse/legacy/api/json.py#L157
@di should I leave the xmlrpc api for release_data alone, or update that as well?
@nixjdm I can't see any reason why it would hurt to add it there as well.
Alright, will do. | 2018-04-25T21:34:35Z | [] | [] |
pypi/warehouse | 3,852 | pypi__warehouse-3852 | [
"3591"
] | 5effea2d3b31698c7434a049d2dc9d91f20537d0 | diff --git a/warehouse/accounts/views.py b/warehouse/accounts/views.py
--- a/warehouse/accounts/views.py
+++ b/warehouse/accounts/views.py
@@ -477,9 +477,9 @@ def profile_callout(user, request):
@view_config(
- route_name="includes.edit-profile-button",
+ route_name="includes.profile-actions",
context=User,
- renderer="includes/accounts/edit-profile-button.html",
+ renderer="includes/accounts/profile-actions.html",
uses_session=True,
)
def edit_profile_button(user, request):
diff --git a/warehouse/routes.py b/warehouse/routes.py
--- a/warehouse/routes.py
+++ b/warehouse/routes.py
@@ -76,8 +76,8 @@ def includeme(config):
domain=warehouse,
)
config.add_route(
- "includes.edit-profile-button",
- "/_includes/edit-profile-button/{username}",
+ "includes.profile-actions",
+ "/_includes/profile-actions/{username}",
factory="warehouse.accounts.models:UserFactory",
traverse="/{username}",
domain=warehouse,
| diff --git a/tests/unit/test_routes.py b/tests/unit/test_routes.py
--- a/tests/unit/test_routes.py
+++ b/tests/unit/test_routes.py
@@ -108,8 +108,8 @@ def add_policy(name, filename):
domain=warehouse,
),
pretend.call(
- "includes.edit-profile-button",
- "/_includes/edit-profile-button/{username}",
+ "includes.profile-actions",
+ "/_includes/profile-actions/{username}",
factory="warehouse.accounts.models:UserFactory",
traverse="/{username}",
domain=warehouse,
| Change 'statistics' text based on whether it is the logged in user
Right now, we have the following text on the user profile page:
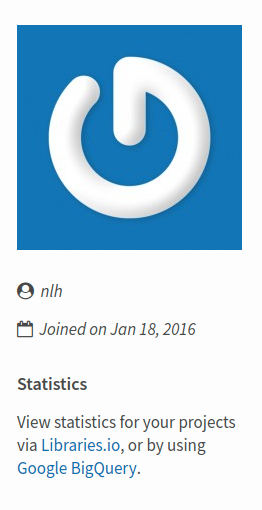
This text shows whether the user being viewed is logged in or not.
What would be better:
1. *If* the logged in user is viewing their *own* public profile, show:
```html
View statistics for your projects via Libraries.io, or by using Google BigQuery.
```
2. *Else* (the user is not logged in, or is viewing the profile of _another_ user), show:
```
View statistics for <username>'s projects via Libraries.io, or by using Google BigQuery.
```
---
**Screenshot Required**: This issue will require an update to the visual design of the site. To help our team give you faster feedback, please include a screenshot in your Pull Request.
| This will be a tad bit tricky, since we are annoyingly strict about access to the session when rendering pages to help in caching. It will either need to be rendered client side, or we'll need to use a client side include for it.
@dstufft - we are already using an include for the 'edit' account button here:
https://github.com/pypa/warehouse/blob/master/warehouse/templates/accounts/profile.html#L35
So, I think it might just be a case of extending (and perhaps renaming?) this file:
https://github.com/pypa/warehouse/blob/master/warehouse/templates/includes/accounts/edit-profile-button.html
@nlhkabu That would work yea, though it'll shift the HTML around a bit since the csi just replaces the content where the csi itself is, it can't replace the content down further. | 2018-04-29T07:28:55Z | [] | [] |
pypi/warehouse | 3,856 | pypi__warehouse-3856 | [
"3845"
] | d652056ec1fad7c2105383397b4732057fe93126 | diff --git a/warehouse/legacy/api/pypi.py b/warehouse/legacy/api/pypi.py
--- a/warehouse/legacy/api/pypi.py
+++ b/warehouse/legacy/api/pypi.py
@@ -118,3 +118,39 @@ def browse(request):
'search', _query={'c': classifier.classifier}
)
)
+
+
+@view_config(route_name='legacy.api.pypi.files')
+def files(request):
+ name = request.params.get('name')
+ version = request.params.get('version')
+
+ if (not name) or (not version):
+ raise HTTPNotFound
+
+ return HTTPMovedPermanently(
+ request.route_path(
+ 'packaging.release', name=name, version=version, _anchor="files"
+ )
+ )
+
+
+@view_config(route_name='legacy.api.pypi.display')
+def display(request):
+ name = request.params.get('name')
+ version = request.params.get('version')
+
+ if not name:
+ raise HTTPNotFound
+
+ if version:
+ return HTTPMovedPermanently(
+ request.route_path(
+ 'packaging.release', name=name, version=version
+ )
+ )
+ return HTTPMovedPermanently(
+ request.route_path(
+ 'packaging.project', name=name
+ )
+ )
diff --git a/warehouse/routes.py b/warehouse/routes.py
--- a/warehouse/routes.py
+++ b/warehouse/routes.py
@@ -289,6 +289,16 @@ def includeme(config):
'browse',
domain=warehouse,
)
+ config.add_pypi_action_route(
+ 'legacy.api.pypi.files',
+ 'files',
+ domain=warehouse,
+ )
+ config.add_pypi_action_route(
+ 'legacy.api.pypi.display',
+ 'display',
+ domain=warehouse,
+ )
# Legacy XMLRPC
config.add_xmlrpc_endpoint(
| diff --git a/tests/unit/legacy/api/test_pypi.py b/tests/unit/legacy/api/test_pypi.py
--- a/tests/unit/legacy/api/test_pypi.py
+++ b/tests/unit/legacy/api/test_pypi.py
@@ -131,3 +131,105 @@ def test_browse_bad_id(self, db_request):
with pytest.raises(HTTPNotFound):
pypi.browse(db_request)
+
+
+class TestFiles:
+
+ def test_files(self, db_request):
+ name = "pip"
+ version = "10.0.0"
+
+ db_request.params = {"name": name, "version": version}
+ db_request.route_path = pretend.call_recorder(
+ lambda *a, **kw: f'/project/{name}/{version}/#files'
+ )
+
+ result = pypi.files(db_request)
+
+ assert isinstance(result, HTTPMovedPermanently)
+ assert result.headers['Location'] == (
+ f'/project/{name}/{version}/#files'
+ )
+ assert result.status_code == 301
+ assert db_request.route_path.calls == [
+ pretend.call(
+ 'packaging.release',
+ name=name,
+ version=version,
+ _anchor="files"
+ )
+ ]
+
+ def test_files_no_version(self, db_request):
+ name = "pip"
+
+ db_request.params = {"name": name}
+
+ with pytest.raises(HTTPNotFound):
+ pypi.files(db_request)
+
+ def test_files_no_name(self, db_request):
+ version = "10.0.0"
+
+ db_request.params = {"version": version}
+
+ with pytest.raises(HTTPNotFound):
+ pypi.files(db_request)
+
+
+class TestDisplay:
+
+ def test_display(self, db_request):
+ name = "pip"
+ version = "10.0.0"
+
+ db_request.params = {"name": name, "version": version}
+ db_request.route_path = pretend.call_recorder(
+ lambda *a, **kw: f'/project/{name}/{version}/'
+ )
+
+ result = pypi.display(db_request)
+
+ assert isinstance(result, HTTPMovedPermanently)
+ assert result.headers['Location'] == (
+ f'/project/{name}/{version}/'
+ )
+ assert result.status_code == 301
+ assert db_request.route_path.calls == [
+ pretend.call(
+ 'packaging.release',
+ name=name,
+ version=version,
+ )
+ ]
+
+ def test_display_no_version(self, db_request):
+ name = "pip"
+
+ db_request.params = {"name": name}
+
+ db_request.route_path = pretend.call_recorder(
+ lambda *a, **kw: f'/project/{name}/'
+ )
+
+ result = pypi.display(db_request)
+
+ assert isinstance(result, HTTPMovedPermanently)
+ assert result.headers['Location'] == (
+ f'/project/{name}/'
+ )
+ assert result.status_code == 301
+ assert db_request.route_path.calls == [
+ pretend.call(
+ 'packaging.project',
+ name=name,
+ )
+ ]
+
+ def test_display_no_name(self, db_request):
+ version = "10.0.0"
+
+ db_request.params = {"version": version}
+
+ with pytest.raises(HTTPNotFound):
+ pypi.display(db_request)
diff --git a/tests/unit/test_routes.py b/tests/unit/test_routes.py
--- a/tests/unit/test_routes.py
+++ b/tests/unit/test_routes.py
@@ -332,6 +332,16 @@ def add_policy(name, filename):
'browse',
domain=warehouse,
),
+ pretend.call(
+ 'legacy.api.pypi.files',
+ 'files',
+ domain=warehouse,
+ ),
+ pretend.call(
+ 'legacy.api.pypi.display',
+ 'display',
+ domain=warehouse,
+ ),
]
assert config.add_pypi_action_redirect.calls == [
| Redirect files &/or display action URLs from legacy
I see some users using legacy URLs like:
* https://pypi.python.org/pypi?:action=files&name=limix&version=1.0.14 (on legacy: https://legacy.pypi.org/pypi?:action=files&name=limix&version=1.0.14 )
* https://pypi.python.org/pypi?:action=display&name=craft-ai (on legacy: https://legacy.pypi.org/pypi?:action=display&name=craft-ai )
* https://pypi.python.org/pypi?:action=display&name=text_progress_bar (on legacy: https://legacy.pypi.org/pypi?:action=display&name=text_progress_bar )
It'd be nice to redirect the display URLs and, potentially, the files URLs to Warehouse.
Followup to #3799.
| Working on this. | 2018-04-30T13:36:21Z | [] | [] |
pypi/warehouse | 3,894 | pypi__warehouse-3894 | [
"3893"
] | b2190fc5404129b6aa775f5f99460d6772a5a6c2 | diff --git a/warehouse/search/tasks.py b/warehouse/search/tasks.py
--- a/warehouse/search/tasks.py
+++ b/warehouse/search/tasks.py
@@ -11,11 +11,15 @@
# limitations under the License.
import binascii
+import urllib
import os
from elasticsearch.helpers import parallel_bulk
+from elasticsearch_dsl import serializer
from sqlalchemy import and_, func
from sqlalchemy.orm import aliased
+import certifi
+import elasticsearch
from warehouse.packaging.models import (
Classifier, Project, Release, release_classifiers)
@@ -98,7 +102,15 @@ def reindex(request):
"""
Recreate the Search Index.
"""
- client = request.registry["elasticsearch.client"]
+ p = urllib.parse.urlparse(request.registry.settings["elasticsearch.url"])
+ client = elasticsearch.Elasticsearch(
+ [urllib.parse.urlunparse(p[:2] + ("",) * 4)],
+ verify_certs=True,
+ ca_certs=certifi.where(),
+ timeout=30,
+ retry_on_timeout=True,
+ serializer=serializer.serializer,
+ )
number_of_replicas = request.registry.get("elasticsearch.replicas", 0)
refresh_interval = request.registry.get("elasticsearch.interval", "1s")
| diff --git a/tests/unit/search/test_tasks.py b/tests/unit/search/test_tasks.py
--- a/tests/unit/search/test_tasks.py
+++ b/tests/unit/search/test_tasks.py
@@ -114,10 +114,17 @@ def project_docs(db):
db_request.registry.update(
{
- "elasticsearch.client": es_client,
"elasticsearch.index": "warehouse",
},
)
+ db_request.registry.settings = {
+ "elasticsearch.url": "http://some.url",
+ }
+ monkeypatch.setattr(
+ warehouse.search.tasks.elasticsearch,
+ "Elasticsearch",
+ lambda *a, **kw: es_client
+ )
class TestException(Exception):
pass
@@ -158,11 +165,18 @@ def project_docs(db):
db_request.registry.update(
{
- "elasticsearch.client": es_client,
"elasticsearch.index": "warehouse",
"elasticsearch.shards": 42,
}
)
+ db_request.registry.settings = {
+ "elasticsearch.url": "http://some.url",
+ }
+ monkeypatch.setattr(
+ warehouse.search.tasks.elasticsearch,
+ "Elasticsearch",
+ lambda *a, **kw: es_client
+ )
parallel_bulk = pretend.call_recorder(lambda client, iterable: [None])
monkeypatch.setattr(
@@ -224,12 +238,19 @@ def project_docs(db):
db_request.registry.update(
{
- "elasticsearch.client": es_client,
"elasticsearch.index": "warehouse",
"elasticsearch.shards": 42,
"sqlalchemy.engine": db_engine,
},
)
+ db_request.registry.settings = {
+ "elasticsearch.url": "http://some.url",
+ }
+ monkeypatch.setattr(
+ warehouse.search.tasks.elasticsearch,
+ "Elasticsearch",
+ lambda *a, **kw: es_client
+ )
parallel_bulk = pretend.call_recorder(lambda client, iterable: [None])
monkeypatch.setattr(
| Tune Elasticsearch client for reindex separately from main search client
#3892 was necessary to alleviate the hard outage experienced due to ES cluster being down.
We were waiting 30s per request for Elasticsearch then retrying. This was added in #1471 to handle reindex issues.
| 2018-05-04T01:45:59Z | [] | [] |
|
pypi/warehouse | 3,899 | pypi__warehouse-3899 | [
"3898"
] | 9374e90fc663a010598a7e0be5cbd89f2e1f42ee | diff --git a/warehouse/manage/views.py b/warehouse/manage/views.py
--- a/warehouse/manage/views.py
+++ b/warehouse/manage/views.py
@@ -594,13 +594,18 @@ def manage_project_roles(project, request, _form_class=CreateRoleForm):
),
)
- owners = (
+ owner_roles = (
request.db.query(Role)
.join(Role.user)
.filter(Role.role_name == 'Owner', Role.project == project)
)
- owner_users = [owner.user for owner in owners]
- owner_users.remove(request.user)
+ owner_users = {owner.user for owner in owner_roles}
+
+ # Don't send to the owner that added the new role
+ owner_users.discard(request.user)
+
+ # Don't send owners email to new user if they are now an owner
+ owner_users.discard(user)
send_collaborator_added_email(
request,
| diff --git a/tests/unit/manage/test_views.py b/tests/unit/manage/test_views.py
--- a/tests/unit/manage/test_views.py
+++ b/tests/unit/manage/test_views.py
@@ -1342,11 +1342,19 @@ def test_post_new_role_validation_fails(self, db_request):
def test_post_new_role(self, monkeypatch, db_request):
project = ProjectFactory.create(name="foobar")
- user = UserFactory.create(username="testuser")
+ new_user = UserFactory.create(username="new_user")
+ owner_1 = UserFactory.create(username="owner_1")
+ owner_2 = UserFactory.create(username="owner_2")
+ owner_1_role = RoleFactory.create(
+ user=owner_1, project=project, role_name="Owner"
+ )
+ owner_2_role = RoleFactory.create(
+ user=owner_2, project=project, role_name="Owner"
+ )
user_service = pretend.stub(
- find_userid=lambda username: user.id,
- get_user=lambda userid: user,
+ find_userid=lambda username: new_user.id,
+ get_user=lambda userid: new_user,
)
db_request.find_service = pretend.call_recorder(
lambda iface, context: user_service
@@ -1354,10 +1362,10 @@ def test_post_new_role(self, monkeypatch, db_request):
db_request.method = "POST"
db_request.POST = pretend.stub()
db_request.remote_addr = "10.10.10.10"
- db_request.user = user
+ db_request.user = owner_1
form_obj = pretend.stub(
validate=pretend.call_recorder(lambda: True),
- username=pretend.stub(data=user.username),
+ username=pretend.stub(data=new_user.username),
role_name=pretend.stub(data="Owner"),
)
form_class = pretend.call_recorder(lambda *a, **kw: form_obj)
@@ -1393,17 +1401,17 @@ def test_post_new_role(self, monkeypatch, db_request):
pretend.call(user_service=user_service),
]
assert db_request.session.flash.calls == [
- pretend.call("Added collaborator 'testuser'", queue="success"),
+ pretend.call("Added collaborator 'new_user'", queue="success"),
]
assert send_collaborator_added_email.calls == [
pretend.call(
db_request,
- user,
+ new_user,
db_request.user,
project.name,
form_obj.role_name.data,
- []
+ {owner_2}
)
]
@@ -1413,23 +1421,27 @@ def test_post_new_role(self, monkeypatch, db_request):
db_request.user,
project.name,
form_obj.role_name.data,
- user,
+ new_user,
),
]
# Only one role is created
- role = db_request.db.query(Role).one()
+ role = db_request.db.query(Role).filter(Role.user == new_user).one()
assert result == {
"project": project,
- "roles_by_user": {user.username: [role]},
+ "roles_by_user": {
+ new_user.username: [role],
+ owner_1.username: [owner_1_role],
+ owner_2.username: [owner_2_role],
+ },
"form": form_obj,
}
entry = db_request.db.query(JournalEntry).one()
assert entry.name == project.name
- assert entry.action == "add Owner testuser"
+ assert entry.action == "add Owner new_user"
assert entry.submitted_by == db_request.user
assert entry.submitted_from == db_request.remote_addr
| Duplicate email when adding a contributor
**Describe the bug**
When I am added as contributor to a project, I get two emails:
* The one telling me I have been added as contributor (good)
* The one telling existing contributors that a new one has been added (bug)
**Expected behavior**
The second email should only be sent to previously existing contributors, otherwise a duplicate is always received by the new contributor (first impressions matter, right now the first thing you see when becoming a contributor is a bug)
**To Reproduce**
Add a new contributor to a project.
**Additional context**
cc @Mariatta #3155
#1000
| 2018-05-04T21:01:44Z | [] | [] |
|
pypi/warehouse | 3,928 | pypi__warehouse-3928 | [
"3914"
] | 5cb55f9969d23bd981ff217a4fd694db0968d824 | diff --git a/warehouse/routes.py b/warehouse/routes.py
--- a/warehouse/routes.py
+++ b/warehouse/routes.py
@@ -330,6 +330,7 @@ def includeme(config):
"/project/{name}/{version}/",
domain=warehouse,
)
+ config.add_redirect("/pypi/", "/", domain=warehouse)
config.add_redirect("/packages/{path:.*}", files_url, domain=warehouse)
# Legacy Action Redirects
| diff --git a/tests/unit/test_routes.py b/tests/unit/test_routes.py
--- a/tests/unit/test_routes.py
+++ b/tests/unit/test_routes.py
@@ -292,6 +292,7 @@ def add_policy(name, filename):
"/project/{name}/{version}/",
domain=warehouse,
),
+ pretend.call("/pypi/", "/", domain=warehouse),
pretend.call(
"/packages/{path:.*}",
"https://files.example.com/packages/{path}",
| Missing legacy redirection from pypi.python.org/pypi/
**Describe the bug**
Redirections from `https://pypi.python.org/pypi/` are not handled (only redirected to `https://pypi.org/pypi/` by varnish (fastly)).
As https://pypi.org/pypi/ does not exists, it creates some broken links.
**Expected behavior**
A 301 to `https://pypi.org/`, simply.
**To Reproduce**
```$ curl -sI https://pypi.python.org/pypi/
HTTP/2 301
server: Varnish
retry-after: 0
location: https://pypi.org/pypi/
[...redacted for readability...]
```
| Per @JulienPalard:
> it's a missing route in warehouse, .... probably in `warehouse/routes.py` around line 327.
I can add this legacy redirect, thanks! | 2018-05-08T20:16:06Z | [] | [] |
pypi/warehouse | 3,974 | pypi__warehouse-3974 | [
"3868"
] | 2c3f81ed46c405bd5d5f6a5f242d482cb2e17b3f | diff --git a/warehouse/views.py b/warehouse/views.py
--- a/warehouse/views.py
+++ b/warehouse/views.py
@@ -276,7 +276,7 @@ def search(request):
# Require match to all specified classifiers
for classifier in request.params.getall("c"):
- query = query.filter("terms", classifiers=[classifier])
+ query = query.query("prefix", classifiers=classifier)
try:
page_num = int(request.params.get("page", 1))
| diff --git a/tests/unit/test_views.py b/tests/unit/test_views.py
--- a/tests/unit/test_views.py
+++ b/tests/unit/test_views.py
@@ -410,6 +410,7 @@ def test_with_classifiers(self, monkeypatch, db_request, metrics, page):
es_query = pretend.stub(
suggest=pretend.call_recorder(lambda *a, **kw: es_query),
filter=pretend.call_recorder(lambda *a, **kw: es_query),
+ query=pretend.call_recorder(lambda *a, **kw: es_query),
sort=pretend.call_recorder(lambda *a, **kw: es_query),
)
db_request.es = pretend.stub(
@@ -460,9 +461,9 @@ def test_with_classifiers(self, monkeypatch, db_request, metrics, page):
assert es_query.suggest.calls == [
pretend.call("name_suggestion", params["q"], term={"field": "name"})
]
- assert es_query.filter.calls == [
- pretend.call("terms", classifiers=["foo :: bar"]),
- pretend.call("terms", classifiers=["fiz :: buz"]),
+ assert es_query.query.calls == [
+ pretend.call("prefix", classifiers="foo :: bar"),
+ pretend.call("prefix", classifiers="fiz :: buz"),
]
assert metrics.histogram.calls == [
pretend.call("warehouse.views.search.results", 1000)
| Unexpected Results on Filtering in Browse by Licence
Given the indentation on the licence tree in the Browse by Licence view I was surprised by the following results:
- Filter on [OSI Approved](https://pypi.org/search/?q=&o=&c=License+%3A%3A+OSI+Approved): **654** Projects
- Add [MIT Filter](https://pypi.org/search/?q=&o=&c=License+%3A%3A+OSI+Approved&c=License+%3A%3A+OSI+Approved+%3A%3A+MIT+License): **95** projects.
- Remove [OSI Approved](https://pypi.org/search/?q=&o=&c=License+%3A%3A+OSI+Approved+%3A%3A+MIT+License): **10,000+** Projects.
Knowing that MIT is OSI Approved, the indentation, _and the inclusion of OSI Approved in the MIT filter URL,_ led me to expect that filtering on OSI Approved was the same as ticking all of the boxes for all of the OSI Approved licences but it seems that instead this box is filtering on packages that explicitly set "OSI Approved" in the meta data and adding MIT, _(or any other),_ restricts this to packages what explicitly set **both** "OSI Approved" **and** "IOS Approved::MIT"!
This is not what I, _and I suspect most people,_ would expect. I do know that really this is the package authors "fault" in that they should have set **both** tags in the meta data but I am not going to raise tickets on over 10, 000 projects and expect a quick resolution.
Possible resolutions:
1. Have a tick box within OSI Approved for Specifically OSI Approved.
2. Any of:
- remove the tick box for OSI Approved and make just a grouping
- remove the grouping
- have toggling OSI Approved (category) toogle ticks on all of the nested lines
- change the filter for OSI Approved (category) from ::OSI Approved to ::OSI Approved* _or whatever the appropriate wildcard is_
- Re-Index the packages so that all packages with any of the OSI Approved licences are automatically included in the OSI Approved category.
| 2018-05-14T18:20:57Z | [] | [] |
|
pypi/warehouse | 3,979 | pypi__warehouse-3979 | [
"3857"
] | 25d94a06d35ac2b654bf60179075a2115b3295f4 | diff --git a/warehouse/csp.py b/warehouse/csp.py
--- a/warehouse/csp.py
+++ b/warehouse/csp.py
@@ -34,6 +34,12 @@ def content_security_policy_tween(request):
except ValueError:
policy = collections.defaultdict(list)
+ # Replace CSP headers on /simple/ pages.
+ if request.path.startswith("/simple/"):
+ policy = collections.defaultdict(list)
+ policy["sandbox"] = ["allow-top-navigation"]
+ policy["default-src"] = [NONE]
+
# We don't want to apply our Content Security Policy to the debug
# toolbar, that's not part of our application and it doesn't work with
# our restrictive CSP.
| diff --git a/tests/unit/test_csp.py b/tests/unit/test_csp.py
--- a/tests/unit/test_csp.py
+++ b/tests/unit/test_csp.py
@@ -163,6 +163,34 @@ def test_devel_csp(self):
"Content-Security-Policy": "script-src https://example.com",
}
+ def test_simple_csp(self):
+ settings = {
+ "csp": {
+ "default-src": ["'none'"],
+ "sandbox": ["allow-top-navigation"],
+ }
+ }
+ response = pretend.stub(headers={})
+ registry = pretend.stub(settings=settings)
+ handler = pretend.call_recorder(lambda request: response)
+
+ tween = csp.content_security_policy_tween_factory(handler, registry)
+
+ request = pretend.stub(
+ scheme="https",
+ host="example.com",
+ path="/simple/",
+ find_service=pretend.call_recorder(
+ lambda *args, **kwargs: settings["csp"],
+ ),
+ )
+
+ assert tween(request) is response
+ assert response.headers == {
+ "Content-Security-Policy":
+ "default-src 'none'; sandbox allow-top-navigation",
+ }
+
class TestCSPPolicy:
def test_create(self):
| use CSP: sandbox on /simple/ pages
https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Content-Security-Policy/sandbox + https://www.youtube.com/watch?v=fbhW37JZtSA&feature=youtu.be
I believe this is a great fit for /simple/, which don't need any ability to do anthing but have a simple HTML structure.
I _think_ we can replace the whole current header with `Content-Security-Policy: sandbox allow-top-navigations`.
| @devd -- I just watched your talk which inspired me to file this. Do you mind weighing in on if the policy I suggested is enough to fully lock down the page (https://pypi.org/simple/cryptography/ is an example of what these pages look like), or do we also need a `default-src: 'none'`?
I think that makes sense. noodling on this, I suspect `default-src: 'none'` is enough but why not both? Good defense in depth.
Thanks @devd!
@dstufft Can you suggest where in the codebase the right place to _override_ the CSP for `/simple/` pages is? | 2018-05-14T20:57:18Z | [] | [] |
pypi/warehouse | 3,980 | pypi__warehouse-3980 | [
"3966"
] | 27cd530101777cc775ffae5d5c1f5a7a1582f2ea | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -52,7 +52,7 @@
JournalEntry,
BlacklistedProject,
)
-from warehouse.utils import http
+from warehouse.utils import http, readme
MAX_FILESIZE = 60 * 1024 * 1024 # 60M
@@ -891,6 +891,33 @@ def file_upload(request):
),
)
+ # Uploading should prevent broken rendered descriptions.
+ if form.description.data:
+ description_content_type = form.description_content_type.data
+ if not description_content_type:
+ description_content_type = "text/x-rst"
+ rendered = readme.render(
+ form.description.data, description_content_type, use_fallback=False
+ )
+ if rendered is None:
+ if form.description_content_type.data:
+ message = (
+ "The description failed to render "
+ "for '{description_content_type}'."
+ ).format(description_content_type=description_content_type)
+ else:
+ message = (
+ "The description failed to render "
+ "in the default format of reStructuredText."
+ )
+ raise _exc_with_message(
+ HTTPBadRequest,
+ "{message} See {projecthelp} for more information.".format(
+ message=message,
+ projecthelp=request.help_url(_anchor="description-content-type"),
+ ),
+ ) from None
+
try:
canonical_version = packaging.utils.canonicalize_version(form.version.data)
release = (
diff --git a/warehouse/utils/readme.py b/warehouse/utils/readme.py
--- a/warehouse/utils/readme.py
+++ b/warehouse/utils/readme.py
@@ -29,7 +29,7 @@
}
-def render(value, content_type=None):
+def render(value, content_type=None, use_fallback=True):
if value is None:
return value
@@ -45,7 +45,8 @@ def render(value, content_type=None):
# If the content was not rendered, we'll render as plaintext instead. The
# reason it's necessary to do this instead of just accepting plaintext is
# that readme_renderer will deal with sanitizing the content.
- if rendered is None:
+ # Skip the fallback option when validating that rendered output is ok.
+ if use_fallback and rendered is None:
rendered = readme_renderer.txt.render(value)
return rendered
| diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -919,6 +919,64 @@ def test_fails_with_invalid_names(self, pyramid_config, db_request, name):
"for more information."
).format(name)
+ @pytest.mark.parametrize(
+ ("description_content_type", "description", "message"),
+ [
+ (
+ "text/x-rst",
+ ".. invalid-directive::",
+ "400 The description failed to render for 'text/x-rst'. "
+ "See /the/help/url/ for more information.",
+ ),
+ (
+ "",
+ ".. invalid-directive::",
+ "400 The description failed to render in the default format "
+ "of reStructuredText. "
+ "See /the/help/url/ for more information.",
+ ),
+ ],
+ )
+ def test_fails_invalid_render(
+ self, pyramid_config, db_request, description_content_type, description, message
+ ):
+ pyramid_config.testing_securitypolicy(userid=1)
+ user = UserFactory.create()
+ EmailFactory.create(user=user)
+ db_request.user = user
+ db_request.remote_addr = "10.10.10.30"
+
+ db_request.POST = MultiDict(
+ {
+ "metadata_version": "1.2",
+ "name": "example",
+ "version": "1.0",
+ "filetype": "sdist",
+ "md5_digest": "a fake md5 digest",
+ "content": pretend.stub(
+ filename="example-1.0.tar.gz",
+ file=io.BytesIO(b"A fake file."),
+ type="application/tar",
+ ),
+ "description_content_type": description_content_type,
+ "description": description,
+ }
+ )
+
+ db_request.help_url = pretend.call_recorder(lambda **kw: "/the/help/url/")
+
+ with pytest.raises(HTTPBadRequest) as excinfo:
+ legacy.file_upload(db_request)
+
+ resp = excinfo.value
+
+ assert db_request.help_url.calls == [
+ pretend.call(_anchor="description-content-type")
+ ]
+
+ assert resp.status_code == 400
+ assert resp.status == message
+
@pytest.mark.parametrize(
"name",
[
@@ -1158,6 +1216,7 @@ def test_successful_upload(
"filetype": "sdist",
"pyversion": "source",
"content": content,
+ "description": "an example description",
}
)
db_request.POST.extend([("classifiers", "Environment :: Other Environment")])
| Fail upload if rST description does not render
If the reStructuredText `long_description` does not render, let's fail the upload instead of just rendering it as plaintext, and raise a meaningful error to the user.
| cc @mblayman
#3760 should help - you can call `warehouse.utils.readme.render` to check and see if the description is valid. | 2018-05-14T21:59:59Z | [] | [] |
pypi/warehouse | 3,988 | pypi__warehouse-3988 | [
"2749",
"3319"
] | d7b6581cc6d3e1e103e558a5a4db169946877645 | diff --git a/warehouse/config.py b/warehouse/config.py
--- a/warehouse/config.py
+++ b/warehouse/config.py
@@ -198,6 +198,7 @@ def configure(settings=None):
maybe_set(settings, "celery.scheduler_url", "REDIS_URL")
maybe_set(settings, "database.url", "DATABASE_URL")
maybe_set(settings, "elasticsearch.url", "ELASTICSEARCH_URL")
+ maybe_set(settings, "elasticsearch.url", "ELASTICSEARCH_SIX_URL")
maybe_set(settings, "sentry.dsn", "SENTRY_DSN")
maybe_set(settings, "sentry.transport", "SENTRY_TRANSPORT")
maybe_set(settings, "sessions.url", "REDIS_URL")
| diff --git a/tests/unit/search/test_tasks.py b/tests/unit/search/test_tasks.py
--- a/tests/unit/search/test_tasks.py
+++ b/tests/unit/search/test_tasks.py
@@ -38,7 +38,7 @@ def test_project_docs(db_session):
assert list(_project_docs(db_session)) == [
{
"_id": p.normalized_name,
- "_type": "project",
+ "_type": "doc",
"_source": {
"created": p.created,
"name": p.name,
| Update elasticsearch-dsl to 6.1.0
There's a new version of [elasticsearch-dsl](https://pypi.python.org/pypi/elasticsearch-dsl) available.
You are currently using **5.4.0**. I have updated it to **6.1.0**
These links might come in handy: <a href="https://pypi.python.org/pypi/elasticsearch-dsl">PyPI</a> | <a href="https://pyup.io/changelogs/elasticsearch-dsl/">Changelog</a> | <a href="https://github.com/elasticsearch/elasticsearch-dsl-py">Repo</a>
### Changelog
>
>### 6.1.0
>------------------
>* Removed ``String`` field.
>* Fixed issue with ``Object``/``Nested`` deserialization
>### 6.0.1
>------------------
>Fixing wheel package for Python 2.7 (803)
>### 6.0.0
>------------------
>Backwards incompatible release compatible with elasticsearch 6.0, changes
>include:
> * use ``doc`` as default ``DocType`` name, this change includes:
> * ``DocType._doc_type.matches`` method is now used to determine which
> ``DocType`` should be used for a hit instead of just checking ``_type``
> * ``Nested`` and ``Object`` field refactoring using newly introduced
> ``InnerDoc`` class. To define a ``Nested``/``Object`` field just define the
> ``InnerDoc`` subclass and then use it when defining the field::
> class Comment(InnerDoc):
> body = Text()
> created_at = Date()
> class Blog(DocType):
> comments = Nested(Comment)
> * methods on ``connections`` singleton are now exposed on the ``connections``
> module directly.
> * field values are now only deserialized when coming from elasticsearch (via
> ``from_es`` method) and not when assigning values in python (either by
> direct assignment or in ``__init__``).
*Got merge conflicts? Close this PR and delete the branch. I'll create a new PR for you.*
Happy merging! 🤖
Update elasticsearch to 6.2.0
This PR updates [elasticsearch](https://pypi.python.org/pypi/elasticsearch) from **5.4.0** to **6.2.0**.
<details>
<summary>Changelog</summary>
### 6.2.0
```
-----------
* Adding Gzip support for capacity constrained networks
* ``_routing`` in bulk action has been deprecated in ES. Introduces a breaking change
if you use ``routing`` as a field in your documents.
```
### 6.1.1
```
------------------
* Updates to SSLContext logic to make it easier to use and have saner defaults.
* Doc updates
```
### 6.1.0
```
------------------
* bad release
```
### 6.0.0
```
------------------
* compatibility with Elasticsearch 6.0.0
```
### 5.6.4
```
* fix handling of UTF-8 surrogates
```
### 5.5.0
```
------------------
* ``streaming_bulk`` helper now supports retries with incremental backoff
* ``scan`` helper properly checks for successful shards instead of just
checking ``failed``
```
</details>
<details>
<summary>Links</summary>
- PyPI: https://pypi.python.org/pypi/elasticsearch
- Changelog: https://pyup.io/changelogs/elasticsearch/
- Repo: https://github.com/elastic/elasticsearch-py
</details>
| 2018-05-15T17:58:42Z | [] | [] |
|
pypi/warehouse | 3,989 | pypi__warehouse-3989 | [
"3746"
] | 359b75e0a4edbd1091b9dd0c2230edbbf5d37c31 | diff --git a/warehouse/search/tasks.py b/warehouse/search/tasks.py
--- a/warehouse/search/tasks.py
+++ b/warehouse/search/tasks.py
@@ -94,7 +94,9 @@ def _project_docs(db):
for release in windowed_query(release_data, Release.name, 50000):
p = ProjectDocType.from_db(release)
p.full_clean()
- yield p.to_dict(include_meta=True)
+ doc = p.to_dict(include_meta=True)
+ doc.pop('_index', None)
+ yield doc
@tasks.task(ignore_result=True, acks_late=True)
@@ -143,7 +145,8 @@ def reindex(request):
try:
request.db.execute("SET statement_timeout = '600s'")
- for _ in parallel_bulk(client, _project_docs(request.db)):
+ for _ in parallel_bulk(client, _project_docs(request.db),
+ index=new_index_name):
pass
except: # noqa
new_index.delete()
| diff --git a/tests/unit/search/test_tasks.py b/tests/unit/search/test_tasks.py
--- a/tests/unit/search/test_tasks.py
+++ b/tests/unit/search/test_tasks.py
@@ -128,9 +128,10 @@ def project_docs(db):
class TestException(Exception):
pass
- def parallel_bulk(client, iterable):
+ def parallel_bulk(client, iterable, index=None):
assert client is es_client
assert iterable is docs
+ assert index == "warehouse-cbcbcbcbcb"
raise TestException
monkeypatch.setattr(
@@ -176,7 +177,9 @@ def project_docs(db):
lambda *a, **kw: es_client
)
- parallel_bulk = pretend.call_recorder(lambda client, iterable: [None])
+ parallel_bulk = pretend.call_recorder(
+ lambda client, iterable, index: [None]
+ )
monkeypatch.setattr(
warehouse.search.tasks, "parallel_bulk", parallel_bulk)
@@ -184,7 +187,9 @@ def project_docs(db):
reindex(db_request)
- assert parallel_bulk.calls == [pretend.call(es_client, docs)]
+ assert parallel_bulk.calls == [
+ pretend.call(es_client, docs, index="warehouse-cbcbcbcbcb")
+ ]
assert es_client.indices.create.calls == [
pretend.call(
body={
@@ -247,7 +252,9 @@ def project_docs(db):
lambda *a, **kw: es_client
)
- parallel_bulk = pretend.call_recorder(lambda client, iterable: [None])
+ parallel_bulk = pretend.call_recorder(
+ lambda client, iterable, index: [None]
+ )
monkeypatch.setattr(
warehouse.search.tasks, "parallel_bulk", parallel_bulk)
@@ -255,7 +262,9 @@ def project_docs(db):
reindex(db_request)
- assert parallel_bulk.calls == [pretend.call(es_client, docs)]
+ assert parallel_bulk.calls == [
+ pretend.call(es_client, docs, index="warehouse-cbcbcbcbcb")
+ ]
assert es_client.indices.create.calls == [
pretend.call(
body={
| Search reindex task leaves empty index.
The 'Search projects' function does not work for me on [https://pypi.org](https://pypi.org). Irrespective of the query, the search does not return any results. (Example: [https://pypi.org/search/?q=numpy](https://pypi.org/search/?q=numpy))
| Me, too.
+1
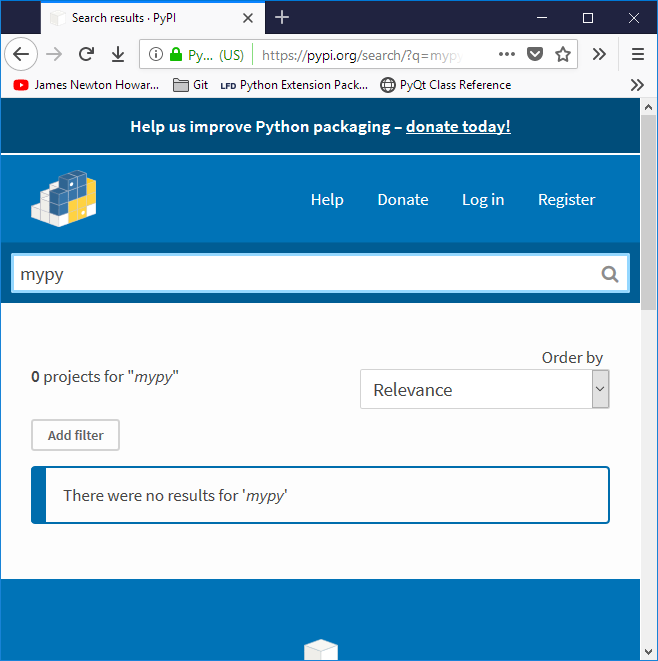
+1
Neither pip 10.0.0 nor pip 9.0.1 showing any results at the moment:
```
pip search pip -v
Starting new HTTPS connection (1): pypi.python.org
https://pypi.python.org:443 "POST /pypi HTTP/1.1" 200 108
```
Same here;
Tested with pip 10, 8, 7
```
$ pip search django -v
Starting new HTTPS connection (1): pypi.python.org
https://pypi.python.org:443 "POST /pypi HTTP/1.1" 200 108
```
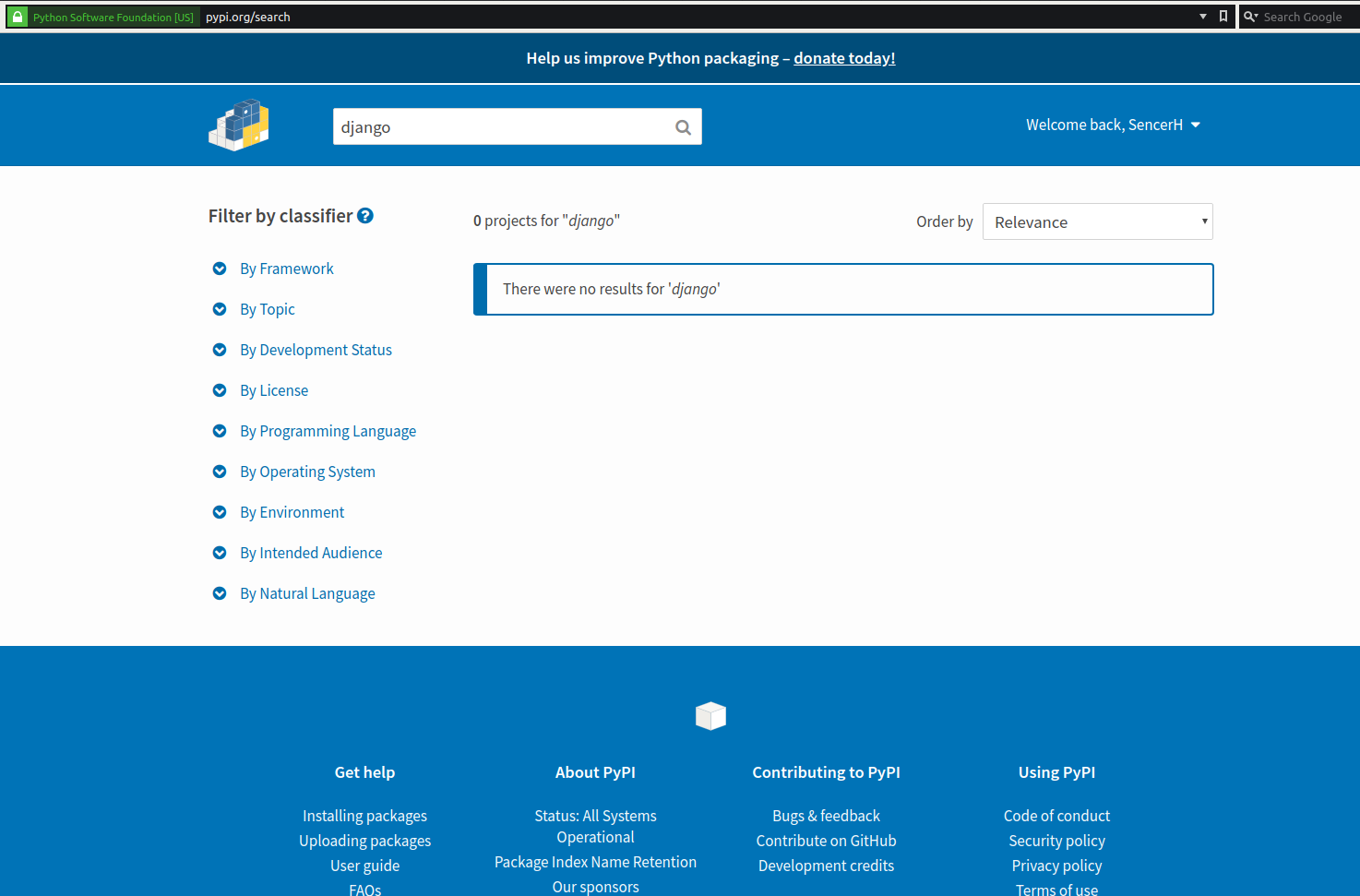
Neither the website search nor pip search returning any results but pip install & pip list -o (which has to query the versions) all seem to be working ok so it looks to be just the search interface.
Same here,
**Critical functionality is broken**!
I'm sure people are already working to solve this
Appears to be solved in web and cli (which probaly use the same endpoint) :+1:
We seem to have some kind of issue in the task that runs every 3 hours to update the index. It was aggravated by changes reverted in #3716, but the underlying issue seems to still be in play.
Something seems to clearly be going wrong in the "swap" in this code: https://github.com/pypa/warehouse/blob/b463af8aac4c778fe5fd1d7abe6e52c00bd06a13/warehouse/search/tasks.py#L131-L167
This seems to be related to running the indexing job as a Celery task. I'm unable to reproduce when running the reindex job from CLI, even kicking two of them off "in competition".
Our ElasticSearch cluster has been upgraded to the latest available release in the 5.x series (5.6.9) from a very early release (5.0).
This was optimistic, aside from being generally a good idea. Perhaps we were hitting some bug that has been resolved.
We also disabled automatic index creation, which _may_ have been leading to the issues encountered leading to #3716.
Aside from this one observation: In our handling of the index swap, we do not wait for a "green" status on the new index before swapping the alias and deleting the old index. Perhaps we should?
Occurred again in prod on the last index task. New index being created, grabbed logs to investigate.
State found:
```
health status index uuid pri rep docs.count docs.deleted store.size pri.store.size
green open production-39b6225ea1 yWEfL5Q6RGu8IlnHvCTvsA 1 2 0 0 486b 162b
```
So the index job attempted to create the new index, but the result was empty. It nevertheless continued on to delete the previous index and take the alias.
logs:
```
[2018-04-19 15:00:00,792: INFO/ForkPoolWorker-5] PUT https://<redacted>.us-east-1.aws.found.io:<redacted>/production-39b6225ea1?wait_for_active_shards=1 [status:200 request:0.712s]
[2018-04-19 15:15:22,702: INFO/ForkPoolWorker-5] POST https://<redacted>.us-east-1.aws.found.io:<redacted>/production-39b6225ea1/_forcemerge [status:200 request:0.020s]
[2018-04-19 15:15:22,786: INFO/ForkPoolWorker-5] PUT https://<redacted>.us-east-1.aws.found.io:<redacted>/production-39b6225ea1/_settings [status:200 request:0.083s]
[2018-04-19 15:15:22,837: INFO/ForkPoolWorker-5] HEAD https://<redacted>.us-east-1.aws.found.io:<redacted>/_alias/production [status:200 request:0.051s]
[2018-04-19 15:15:22,854: INFO/ForkPoolWorker-5] GET https://<redacted>.us-east-1.aws.found.io:<redacted>/_alias/production [status:200 request:0.017s]
[2018-04-19 15:15:23,067: INFO/ForkPoolWorker-5] POST https://<redacted>.us-east-1.aws.found.io:<redacted>/_aliases [status:200 request:0.213s]
[2018-04-19 15:15:23,682: INFO/ForkPoolWorker-5] DELETE https://<redacted>.us-east-1.aws.found.io:<redacted>/production-c7d6538b0d [status:200 request:0.614s]
```
excluded _bulk calls for clarity, but there were plenty of them!
```
grep <redacted> worker-recent | grep '2018-04-19 15:' | grep _bulk | wc
270 2430 54810
```
comparing to two previous runs:
```
grep <redacted> worker-recent | grep '2018-04-19 12' | grep _bulk | wc
274 2466 55641
grep <redacted> worker-recent | grep '2018-04-19 09' | grep _bulk | wc
269 2421 54607
```
it seems #3774 may have helped... which leads me to believe some state was being cached by the celery worker...
haven't had any issues since #3774 deployed... continuing to keep an eye on things. added metric for search result counts in #3772 to alert us when index is empty.
we've been going steady for 3 days. closing.
The problem is that `Project` doesn't have the new `index` associated with it when running in celery - the `Index.doc_type` method only sets the `index` name on the `DocType` *if no index is set*. That would explain why it runs fine from CLI but not from celery - CLI starts with fresh objects that don't have an index associated with it while celery is a long running process where it might happen that the previous `reindex` run has already registered an index name with the `Project` doc type. Should be a simple fix, PR incoming | 2018-05-15T18:01:52Z | [] | [] |
pypi/warehouse | 4,015 | pypi__warehouse-4015 | [
"4012"
] | 2e6dc9856ed600071e912dd237b446500ff4f543 | diff --git a/warehouse/routes.py b/warehouse/routes.py
--- a/warehouse/routes.py
+++ b/warehouse/routes.py
@@ -325,17 +325,6 @@ def includeme(config):
# Legacy Redirects
config.add_redirect("/pypi/{name}/", "/project/{name}/", domain=warehouse)
- config.add_redirect(
- "/pypi/{name}/{version}/json/",
- "/pypi/{name}/{version}/json",
- domain=warehouse,
- )
- config.add_redirect(
- "/pypi/{name}/json/",
- "/pypi/{name}/json",
- domain=warehouse,
- )
-
config.add_redirect(
"/pypi/{name}/{version}/",
"/project/{name}/{version}/",
| diff --git a/tests/unit/test_routes.py b/tests/unit/test_routes.py
--- a/tests/unit/test_routes.py
+++ b/tests/unit/test_routes.py
@@ -287,16 +287,6 @@ def add_policy(name, filename):
assert config.add_redirect.calls == [
pretend.call("/p/{name}/", "/project/{name}/", domain=warehouse),
pretend.call("/pypi/{name}/", "/project/{name}/", domain=warehouse),
- pretend.call(
- "/pypi/{name}/{version}/json/",
- "/pypi/{name}/{version}/json",
- domain=warehouse,
- ),
- pretend.call(
- "/pypi/{name}/json/",
- "/pypi/{name}/json",
- domain=warehouse,
- ),
pretend.call(
"/pypi/{name}/{version}/",
"/project/{name}/{version}/",
| Redirect loop when requesting missing package
**Describe the bug**
When I `curl -v https://pypi.org/pypi/bogus/1.0.0/json` with an invalid package/version combination, I get put in an infinite redirect loop. If the package/version combination is correct, then the data is served as expected.
I noticed this because the `license_finder` gem just now started having issues when looking for my team's internal (non-PyPi) packages. Previously, it hadn't complained.
**Expected behavior**
For an invalid package/version combination, I'd expect a 404 (or whatever the previous behavior was).
**To Reproduce**
`curl -v https://pypi.org/pypi/bogus/1.0.0/json`, note that the `Location` header leads you to the same URL.
**My Platform**
Pretty sure my platform isn't relevant. I've reproduced the problem both from my personal internet connection as well as within AWS.
**Additional context**
Adding @dstufft @di as suggested on IRC.
| I'm guessing this is likely fallout from https://github.com/pypa/warehouse/pull/3971.
Looks like this is because we use `@notfound_view_config(append_slash=HTTPMovedPermanently)` with our exception view, so when it's not found, it gets a redirect to the same URL with the trailing slash. | 2018-05-16T20:32:15Z | [] | [] |
pypi/warehouse | 4,184 | pypi__warehouse-4184 | [
"3831"
] | 53e9235a3ac835c17b82433186d4d898caa6e46e | diff --git a/warehouse/legacy/api/json.py b/warehouse/legacy/api/json.py
--- a/warehouse/legacy/api/json.py
+++ b/warehouse/legacy/api/json.py
@@ -10,6 +10,8 @@
# See the License for the specific language governing permissions and
# limitations under the License.
+from collections import OrderedDict
+
from pyramid.httpexceptions import HTTPMovedPermanently, HTTPNotFound
from pyramid.view import view_config
from sqlalchemy.orm import Load
@@ -161,6 +163,7 @@ def json_release(release, request):
"downloads": {"last_day": -1, "last_week": -1, "last_month": -1},
"package_url": request.route_url("packaging.project", name=project.name),
"project_url": request.route_url("packaging.project", name=project.name),
+ "project_urls": OrderedDict(release.urls) if release.urls else None,
"release_url": request.route_url(
"packaging.release", name=project.name, version=release.version
),
| diff --git a/tests/unit/legacy/api/test_json.py b/tests/unit/legacy/api/test_json.py
--- a/tests/unit/legacy/api/test_json.py
+++ b/tests/unit/legacy/api/test_json.py
@@ -10,11 +10,14 @@
# See the License for the specific language governing permissions and
# limitations under the License.
+from collections import OrderedDict
+
import pretend
from pyramid.httpexceptions import HTTPMovedPermanently, HTTPNotFound
from warehouse.legacy.api import json
+from warehouse.packaging.models import Dependency, DependencyKind
from ....common.db.accounts import UserFactory
from ....common.db.packaging import (
@@ -138,9 +141,27 @@ def test_normalizing_redirects(self, db_request):
pretend.call(name=release.project.name)
]
- def test_detail_renders(self, pyramid_config, db_request):
+ def test_detail_renders(self, pyramid_config, db_request, db_session):
project = ProjectFactory.create(has_docs=True)
description_content_type = "text/x-rst"
+ url = "/the/fake/url/"
+ project_urls = [
+ "url," + url,
+ "Homepage,https://example.com/home2/",
+ "Source Code,https://example.com/source-code/",
+ "uri,http://[email protected]:123/forum/questions/?tag=networking&order=newest#top", # noqa: E501
+ "ldap,ldap://[2001:db8::7]/c=GB?objectClass?one",
+ "tel,tel:+1-816-555-1212",
+ "telnet,telnet://192.0.2.16:80/",
+ "urn,urn:oasis:names:specification:docbook:dtd:xml:4.1.2",
+ "reservedchars,http://example.com?&$+/:;=@#", # Commas don't work!
+ "unsafechars,http://example.com <>[]{}|\^%",
+ ]
+ expected_urls = []
+ for project_url in reversed(project_urls):
+ expected_urls.append(tuple(project_url.split(",")))
+ expected_urls = OrderedDict(tuple(expected_urls))
+
releases = [
ReleaseFactory.create(project=project, version=v)
for v in ["0.1", "1.0", "2.0"]
@@ -153,6 +174,16 @@ def test_detail_renders(self, pyramid_config, db_request):
)
]
+ for urlspec in project_urls:
+ db_session.add(
+ Dependency(
+ name=releases[3].project.name,
+ version="3.0",
+ kind=DependencyKind.project_url.value,
+ specifier=urlspec,
+ )
+ )
+
files = [
FileFactory.create(
release=r,
@@ -167,7 +198,6 @@ def test_detail_renders(self, pyramid_config, db_request):
JournalEntryFactory.reset_sequence()
je = JournalEntryFactory.create(name=project.name, submitted_by=user)
- url = "/the/fake/url/"
db_request.route_url = pretend.call_recorder(lambda *args, **kw: url)
result = json.json_release(releases[3], db_request)
@@ -203,9 +233,10 @@ def test_detail_renders(self, pyramid_config, db_request):
"maintainer": None,
"maintainer_email": None,
"name": project.name,
+ "package_url": "/the/fake/url/",
"platform": None,
"project_url": "/the/fake/url/",
- "package_url": "/the/fake/url/",
+ "project_urls": expected_urls,
"release_url": "/the/fake/url/",
"requires_dist": None,
"requires_python": None,
@@ -344,9 +375,10 @@ def test_minimal_renders(self, pyramid_config, db_request):
"maintainer": None,
"maintainer_email": None,
"name": project.name,
+ "package_url": "/the/fake/url/",
"platform": None,
"project_url": "/the/fake/url/",
- "package_url": "/the/fake/url/",
+ "project_urls": None,
"release_url": "/the/fake/url/",
"requires_dist": None,
"requires_python": None,
| Expose project_urls in JSON API
https://packaging.python.org/tutorials/distributing-packages/#project-urls
Related to #3798 / #3820
I realized project_urls is not currently exposed by the JSON API. I propose adding it.
Though the keys in the project_urls dict can be anything, they're fairly standardized, enough to be useful when querying for them over and API. For example, [Flask's API response](https://pypi.org/pypi/Flask/json) lists its home_page as https://www.palletsprojects.com/p/flask/ (not it's github account which is fairly typical), and puts it's GitHub link in `project_urls['Code']`, which is not currently in the API response.
| @nixjdm Shall I assign this to you?
Sure :) It's so similar it'd be no problem, especially after the other PR get's in.
@nixjdm I was wondering how work was going on this feature? | 2018-06-15T18:07:27Z | [] | [] |
pypi/warehouse | 4,274 | pypi__warehouse-4274 | [
"4273"
] | 43ff0a7696fe47ab42d94ca7dea7f234ed2911d9 | diff --git a/warehouse/email/services.py b/warehouse/email/services.py
--- a/warehouse/email/services.py
+++ b/warehouse/email/services.py
@@ -11,7 +11,9 @@
# limitations under the License.
from email.headerregistry import Address
-from email.utils import parseaddr
+from email.mime.multipart import MIMEMultipart
+from email.mime.text import MIMEText
+from email.utils import parseaddr, formataddr
from pyramid_mailer import get_mailer
from pyramid_mailer.message import Message
@@ -68,13 +70,25 @@ def create_service(cls, context, request):
)
def send(self, subject, body, *, recipient):
- resp = self._client.send_email(
+ message = MIMEMultipart("mixed")
+ message["Subject"] = subject
+ message["From"] = self._sender
+
+ # The following is necessary to support friendly names with Unicode characters,
+ # otherwise the entire value will get encoded and will not be accepted by SES:
+ #
+ # >>> parseaddr("Fööbar <[email protected]>")
+ # ('Fööbar', '[email protected]')
+ # >>> formataddr(_)
+ # '=?utf-8?b?RsO2w7ZiYXI=?= <[email protected]>'
+ message["To"] = formataddr(parseaddr(recipient))
+
+ message.attach(MIMEText(body, "plain", "utf-8"))
+
+ resp = self._client.send_raw_email(
Source=self._sender,
- Destination={"ToAddresses": [recipient]},
- Message={
- "Subject": {"Data": subject, "Charset": "UTF-8"},
- "Body": {"Text": {"Data": body, "Charset": "UTF-8"}},
- },
+ Destinations=[recipient],
+ RawMessage={"Data": message.as_string()},
)
self._db.add(
| diff --git a/tests/unit/email/test_services.py b/tests/unit/email/test_services.py
--- a/tests/unit/email/test_services.py
+++ b/tests/unit/email/test_services.py
@@ -102,9 +102,17 @@ def test_creates_service(self):
assert sender._db is request.db
def test_send(self, db_session):
+ # Determine what the random boundary token will be
+ import random
+ import sys
+
+ random.seed(42)
+ token = random.randrange(sys.maxsize)
+ random.seed(42)
+
resp = {"MessageId": str(uuid.uuid4()) + "-ses"}
aws_client = pretend.stub(
- send_email=pretend.call_recorder(lambda *a, **kw: resp)
+ send_raw_email=pretend.call_recorder(lambda *a, **kw: resp)
)
sender = SESEmailSender(
aws_client, sender="DevPyPI <[email protected]>", db=db_session
@@ -116,13 +124,27 @@ def test_send(self, db_session):
recipient="FooBar <[email protected]>",
)
- assert aws_client.send_email.calls == [
+ assert aws_client.send_raw_email.calls == [
pretend.call(
Source="DevPyPI <[email protected]>",
- Destination={"ToAddresses": ["FooBar <[email protected]>"]},
- Message={
- "Subject": {"Data": "This is a Subject", "Charset": "UTF-8"},
- "Body": {"Text": {"Data": "This is a Body", "Charset": "UTF-8"}},
+ Destinations=["FooBar <[email protected]>"],
+ RawMessage={
+ "Data": (
+ 'Content-Type: multipart/mixed; boundary="==============={token}=="\n' # noqa
+ "MIME-Version: 1.0\n"
+ "Subject: This is a Subject\n"
+ "From: DevPyPI <[email protected]>\n"
+ "To: FooBar <[email protected]>\n"
+ "\n"
+ "--==============={token}==\n"
+ 'Content-Type: text/plain; charset="utf-8"\n'
+ "MIME-Version: 1.0\n"
+ "Content-Transfer-Encoding: base64\n"
+ "\n"
+ "VGhpcyBpcyBhIEJvZHk=\n"
+ "\n"
+ "--==============={token}==--\n"
+ ).format(token=token)
},
)
]
| PyPI sends emails with unicode in header
**Describe the bug**
<!-- A clear and concise description the bug -->
I created a new account on pypi.org, but I am not receiving any email verification link by email - not after accouunt creation and also not after resending the verification link (even if pypi.org claims "Verification email for [email protected] resent").
test.pypi.org works for me, using the same account name and email address. I deleted and recreated the account on both pypi.org and test.pypi.org - same behaviour (well, the initial email did sometimes not arrive on test.pypi.org, but the the verification resend always arrived right away).
**Expected behavior**
<!-- A clear and concise description of what you expected to happen -->
I should get an email token after registering, and also when I resend the verification mail from the account page. I know it might take a while, but I am experiencing this since Friday.
**To Reproduce**
<!-- Steps to reproduce the bug, or a link to PyPI where the bug is visible -->
Register on pypi.org, wait for email. Resend the verification email from the account settings page, wait again.
**My Platform**
<!--
Any details about your specific platform:
* If the problem is in the browser, what browser, version, and OS?
* If the problem is with a command-line tool, what version of that tool?
* If the problem is with connecting to PyPI, include some details about
your network, including SSL/TLS implementation in use, internet service
provider, and if there are any firewalls or proxies in use.
-->
Firefox 52
| Hello @nhoening! PyPI received bounces for the address you specified which led to it being placed on the suppression list.
I've removed it from the suppression list, can you try again?
Yes, that did it. Thanks for the quick help!
@nhoening in taking a closer look, @di noticed that your domain's mail transfer agent was rejecting the `To` field due to the ö in your last name:
```
emailAddress: Nicolas Höning <[email protected]>
diagnosticCode: smtp; 550 To contains invalid characters.
```
Warehouse constructs `To` headers using:
https://github.com/pypa/warehouse/blob/40fbc32fef7c7ffe41cd18f3f8951578555db1aa/warehouse/email/__init__.py#L25-L33
So it appears that either:
1) Your mail transfer agent no supports unicode
2) You temporarily changed your profile display name, as it now contains the ö character
Can you confirm if either of these occurred? I may be imagining things, but I briefly recall seeing the non-unicode "variant" of your name in your profile when taking a look initially.
My concern is that future email may not be deliverable.
I can confirm that I tried to rule out the umlaut as a problem. I had used it initially and after a day or two, I went on testing without the umlaut. test.pypi.org also did not work initially, until I removed it, but I thought it must be a glitch. It makes more sense now.
So, either I should talk to my email provider, or you guys decide that [RFC-5322 Article 2.2](https://tools.ietf.org/html/rfc5322#section-2.22) (see below) applies to this problem (I wouldn't be expert enough to tell) and maybe encode header text.
> Header fields are lines beginning with a field name, followed by a
> colon (":"), followed by a field body, and terminated by CRLF. A
> field name MUST be composed of printable US-ASCII characters (i.e.,
> characters that have values between 33 and 126, inclusive), except
> colon. A field body may be composed of printable US-ASCII characters
> as well as the space (SP, ASCII value 32) and horizontal tab (HTAB,
> ASCII value 9) characters (together known as the white space
> characters, WSP).
Reopening this because I think it's something that can be fixed on our end. | 2018-07-09T18:29:12Z | [] | [] |
pypi/warehouse | 4,325 | pypi__warehouse-4325 | [
"3024"
] | da24703a943bf5fda22999955e724258960516a2 | diff --git a/warehouse/legacy/api/json.py b/warehouse/legacy/api/json.py
--- a/warehouse/legacy/api/json.py
+++ b/warehouse/legacy/api/json.py
@@ -138,6 +138,7 @@ def json_release(release, request):
"downloads": -1,
"upload_time": f.upload_time.strftime("%Y-%m-%dT%H:%M:%S"),
"url": request.route_url("packaging.file", path=f.path),
+ "requires_python": r.requires_python if r.requires_python else None,
}
for f in fs
]
| diff --git a/tests/unit/legacy/api/test_json.py b/tests/unit/legacy/api/test_json.py
--- a/tests/unit/legacy/api/test_json.py
+++ b/tests/unit/legacy/api/test_json.py
@@ -263,6 +263,7 @@ def test_detail_renders(self, pyramid_config, db_request, db_session):
"%Y-%m-%dT%H:%M:%S"
),
"url": "/the/fake/url/",
+ "requires_python": None,
}
],
"2.0": [
@@ -283,6 +284,7 @@ def test_detail_renders(self, pyramid_config, db_request, db_session):
"%Y-%m-%dT%H:%M:%S"
),
"url": "/the/fake/url/",
+ "requires_python": None,
}
],
"3.0": [
@@ -303,6 +305,7 @@ def test_detail_renders(self, pyramid_config, db_request, db_session):
"%Y-%m-%dT%H:%M:%S"
),
"url": "/the/fake/url/",
+ "requires_python": None,
}
],
},
@@ -322,6 +325,7 @@ def test_detail_renders(self, pyramid_config, db_request, db_session):
"size": 200,
"upload_time": files[2].upload_time.strftime("%Y-%m-%dT%H:%M:%S"),
"url": "/the/fake/url/",
+ "requires_python": None,
}
],
"last_serial": je.id,
@@ -402,6 +406,7 @@ def test_minimal_renders(self, pyramid_config, db_request):
"size": 200,
"upload_time": file.upload_time.strftime("%Y-%m-%dT%H:%M:%S"),
"url": "/the/fake/url/",
+ "requires_python": None,
}
]
},
@@ -418,6 +423,7 @@ def test_minimal_renders(self, pyramid_config, db_request):
"size": 200,
"upload_time": file.upload_time.strftime("%Y-%m-%dT%H:%M:%S"),
"url": "/the/fake/url/",
+ "requires_python": None,
}
],
"last_serial": je.id,
| Expose requires_python for each release in JSON API
The following JSON:
https://pypi.python.org/pypi/astropy/json
or:
https://pypi.io/pypi/astropy/json
includes the following for the latest release:
```
"requires_python": ">=3.5",
```
but it would be really helpful to have this for all releases listed in the ``releases`` section, to avoid having to hit the server for each release to find out the ``requires_python``.
| Thanks for your suggestion, and sorry for the slow response! I like this idea and am grateful to you for bringing it up.
The folks working on Warehouse have gotten [funding to concentrate on improving and deploying Warehouse](https://pyfound.blogspot.com/2017/11/the-psf-awarded-moss-grant-pypi.html), and are concentrating on work towards [our development roadmap](https://wiki.python.org/psf/WarehouseRoadmap) -- the most urgent task is to improve Warehouse to the point where we can redirect pypi.python.org to pypi.org so the site is more sustainable and reliable. Since (as far as I can gather) this feature isn't something that the legacy site has, I've moved it to a future milestone.
Thanks and sorry again for the wait.
Just noting that the current bulk API for accessing the `Requires-Python` metadata is PEP 503's `data-requires-python` link attribute: https://www.python.org/dev/peps/pep-0503/#specification
For AstroPy specifically, that appears at https://pypi.org/simple/astropy/ and https://pypi.python.org/simple/astropy/
Having a project level JSON listing *would* still be a nice improvement, but in the meantime, querying the link attributes in the PEP 503 API is preferred to querying each release individually. | 2018-07-19T23:38:48Z | [] | [] |
pypi/warehouse | 4,405 | pypi__warehouse-4405 | [
"4022"
] | ee3ce4247bf569edf8cbf0e1b12fef96ed71d0f7 | diff --git a/warehouse/admin/routes.py b/warehouse/admin/routes.py
--- a/warehouse/admin/routes.py
+++ b/warehouse/admin/routes.py
@@ -66,6 +66,20 @@ def includeme(config):
traverse="/{project_name}",
domain=warehouse,
)
+ config.add_route(
+ "admin.project.add_role",
+ "/admin/projects/{project_name}/add_role/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{project_name}",
+ domain=warehouse,
+ )
+ config.add_route(
+ "admin.project.delete_role",
+ "/admin/projects/{project_name}/delete_role/{role_id}/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{project_name}",
+ domain=warehouse,
+ )
config.add_route(
"admin.project.delete",
"/admin/projects/{project_name}/delete/",
diff --git a/warehouse/admin/views/projects.py b/warehouse/admin/views/projects.py
--- a/warehouse/admin/views/projects.py
+++ b/warehouse/admin/views/projects.py
@@ -16,6 +16,7 @@
from pyramid.httpexceptions import HTTPBadRequest, HTTPMovedPermanently, HTTPSeeOther
from pyramid.view import view_config
from sqlalchemy import or_
+from sqlalchemy.orm.exc import NoResultFound
from warehouse.accounts.models import User
from warehouse.packaging.models import Project, Release, Role, JournalEntry
@@ -271,6 +272,125 @@ def set_upload_limit(project, request):
)
+@view_config(
+ route_name="admin.project.add_role",
+ permission="admin",
+ request_method="POST",
+ uses_session=True,
+ require_methods=False,
+)
+def add_role(project, request):
+ username = request.POST.get("username")
+ if not username:
+ request.session.flash("Provide a username", queue="error")
+ raise HTTPSeeOther(
+ request.route_path(
+ "admin.project.detail", project_name=project.normalized_name
+ )
+ )
+
+ try:
+ user = request.db.query(User).filter(User.username == username).one()
+ except NoResultFound:
+ request.session.flash(f"Unknown username '{username}'", queue="error")
+ raise HTTPSeeOther(
+ request.route_path(
+ "admin.project.detail", project_name=project.normalized_name
+ )
+ )
+
+ role_name = request.POST.get("role_name")
+ if not role_name:
+ request.session.flash("Provide a role", queue="error")
+ raise HTTPSeeOther(
+ request.route_path(
+ "admin.project.detail", project_name=project.normalized_name
+ )
+ )
+
+ already_there = (
+ request.db.query(Role)
+ .filter(Role.user == user, Role.project == project)
+ .count()
+ )
+
+ if already_there > 0:
+ request.session.flash(
+ f"User '{user.username}' already has a role on this project", queue="error"
+ )
+ raise HTTPSeeOther(
+ request.route_path(
+ "admin.project.detail", project_name=project.normalized_name
+ )
+ )
+
+ request.db.add(
+ JournalEntry(
+ name=project.name,
+ action=f"add {role_name} {user.username}",
+ submitted_by=request.user,
+ submitted_from=request.remote_addr,
+ )
+ )
+
+ request.db.add(Role(role_name=role_name, user=user, project=project))
+
+ request.session.flash(
+ f"Added '{user.username}' as '{role_name}' on '{project.name}'", queue="success"
+ )
+ return HTTPSeeOther(
+ request.route_path("admin.project.detail", project_name=project.normalized_name)
+ )
+
+
+@view_config(
+ route_name="admin.project.delete_role",
+ permission="admin",
+ request_method="POST",
+ uses_session=True,
+ require_methods=False,
+)
+def delete_role(project, request):
+ confirm = request.POST.get("username")
+ role_id = request.matchdict.get("role_id")
+
+ role = request.db.query(Role).get(role_id)
+ if not role:
+ request.session.flash(f"This role no longer exists", queue="error")
+ raise HTTPSeeOther(
+ request.route_path(
+ "admin.project.detail", project_name=project.normalized_name
+ )
+ )
+
+ if not confirm or confirm != role.user_name:
+ request.session.flash("Confirm the request", queue="error")
+ raise HTTPSeeOther(
+ request.route_path(
+ "admin.project.detail", project_name=project.normalized_name
+ )
+ )
+
+ request.session.flash(
+ f"Removed '{role.user_name}' as '{role.role_name}' on '{project.name}'",
+ queue="success",
+ )
+ request.db.add(
+ JournalEntry(
+ name=project.name,
+ action=f"remove {role.role_name} {role.user_name}",
+ submitted_by=request.user,
+ submitted_from=request.remote_addr,
+ )
+ )
+
+ request.db.delete(role)
+
+ return HTTPSeeOther(
+ request.route_path("admin.project.detail", project_name=project.normalized_name)
+ )
+
+
@view_config(
route_name="admin.project.delete",
permission="admin",
| diff --git a/tests/unit/admin/test_routes.py b/tests/unit/admin/test_routes.py
--- a/tests/unit/admin/test_routes.py
+++ b/tests/unit/admin/test_routes.py
@@ -69,6 +69,20 @@ def test_includeme():
traverse="/{project_name}",
domain=warehouse,
),
+ pretend.call(
+ "admin.project.add_role",
+ "/admin/projects/{project_name}/add_role/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{project_name}",
+ domain=warehouse,
+ ),
+ pretend.call(
+ "admin.project.delete_role",
+ "/admin/projects/{project_name}/delete_role/{role_id}/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{project_name}",
+ domain=warehouse,
+ ),
pretend.call(
"admin.project.delete",
"/admin/projects/{project_name}/delete/",
diff --git a/tests/unit/admin/views/test_projects.py b/tests/unit/admin/views/test_projects.py
--- a/tests/unit/admin/views/test_projects.py
+++ b/tests/unit/admin/views/test_projects.py
@@ -12,11 +12,12 @@
import pretend
import pytest
+import uuid
from pyramid.httpexceptions import HTTPBadRequest, HTTPMovedPermanently, HTTPSeeOther
from warehouse.admin.views import projects as views
-from warehouse.packaging.models import Project
+from warehouse.packaging.models import Project, Role
from ....common.db.accounts import UserFactory
from ....common.db.packaging import (
@@ -431,3 +432,164 @@ def test_deletes_project(self, db_request):
]
assert not (db_request.db.query(Project).filter(Project.name == "foo").count())
+
+
+class TestAddRole:
+ def test_add_role(self, db_request):
+ role_name = "Maintainer"
+ project = ProjectFactory.create(name="foo")
+ user = UserFactory.create(username="bar")
+
+ db_request.route_path = pretend.call_recorder(lambda *a, **kw: "/the-redirect/")
+ db_request.session = pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None)
+ )
+ db_request.POST["username"] = user.username
+ db_request.POST["role_name"] = role_name
+ db_request.user = UserFactory.create()
+ db_request.remote_addr = "192.168.1.1"
+
+ views.add_role(project, db_request)
+
+ assert db_request.session.flash.calls == [
+ pretend.call(f"Added 'bar' as '{role_name}' on 'foo'", queue="success")
+ ]
+
+ role = db_request.db.query(Role).one()
+ assert role.role_name == role_name
+ assert role.user == user
+ assert role.project == project
+
+ def test_add_role_no_username(self, db_request):
+ project = ProjectFactory.create(name="foo")
+
+ db_request.POST = {}
+ db_request.route_path = pretend.call_recorder(lambda *a, **kw: "/the-redirect/")
+ db_request.session = pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None)
+ )
+
+ with pytest.raises(HTTPSeeOther):
+ views.add_role(project, db_request)
+
+ assert db_request.session.flash.calls == [
+ pretend.call(f"Provide a username", queue="error")
+ ]
+
+ def test_add_role_no_user(self, db_request):
+ project = ProjectFactory.create(name="foo")
+
+ db_request.POST = {"username": "bar"}
+ db_request.route_path = pretend.call_recorder(lambda *a, **kw: "/the-redirect/")
+ db_request.session = pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None)
+ )
+
+ with pytest.raises(HTTPSeeOther):
+ views.add_role(project, db_request)
+
+ assert db_request.session.flash.calls == [
+ pretend.call(f"Unknown username 'bar'", queue="error")
+ ]
+
+ def test_add_role_no_role_name(self, db_request):
+ project = ProjectFactory.create(name="foo")
+ UserFactory.create(username="bar")
+
+ db_request.POST = {"username": "bar"}
+ db_request.route_path = pretend.call_recorder(lambda *a, **kw: "/the-redirect/")
+ db_request.session = pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None)
+ )
+
+ with pytest.raises(HTTPSeeOther):
+ views.add_role(project, db_request)
+
+ assert db_request.session.flash.calls == [
+ pretend.call(f"Provide a role", queue="error")
+ ]
+
+ def test_add_role_with_existing_role(self, db_request):
+ project = ProjectFactory.create(name="foo")
+ user = UserFactory.create(username="bar")
+ role = RoleFactory.create(project=project, user=user)
+
+ db_request.POST = {"username": "bar", "role_name": role.role_name}
+ db_request.route_path = pretend.call_recorder(lambda *a, **kw: "/the-redirect/")
+ db_request.session = pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None)
+ )
+
+ with pytest.raises(HTTPSeeOther):
+ views.add_role(project, db_request)
+
+ assert db_request.session.flash.calls == [
+ pretend.call(
+ f"User 'bar' already has a role on this project", queue="error"
+ )
+ ]
+
+
+class TestDeleteRole:
+ def test_delete_role(self, db_request):
+ project = ProjectFactory.create(name="foo")
+ user = UserFactory.create(username="bar")
+ role = RoleFactory.create(project=project, user=user)
+
+ db_request.route_path = pretend.call_recorder(lambda *a, **kw: "/the-redirect/")
+ db_request.session = pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None)
+ )
+ db_request.POST["username"] = user.username
+ db_request.matchdict["role_id"] = role.id
+ db_request.user = UserFactory.create()
+ db_request.remote_addr = "192.168.1.1"
+
+ views.delete_role(project, db_request)
+
+ assert db_request.session.flash.calls == [
+ pretend.call(
+ f"Removed '{role.user_name}' as '{role.role_name}' on '{project.name}'",
+ queue="success",
+ )
+ ]
+
+ assert db_request.db.query(Role).all() == []
+
+ def test_delete_role_not_found(self, db_request):
+ project = ProjectFactory.create(name="foo")
+
+ db_request.route_path = pretend.call_recorder(lambda *a, **kw: "/the-redirect/")
+ db_request.session = pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None)
+ )
+ db_request.matchdict["role_id"] = uuid.uuid4()
+ db_request.user = UserFactory.create()
+ db_request.remote_addr = "192.168.1.1"
+
+ with pytest.raises(HTTPSeeOther):
+ views.delete_role(project, db_request)
+
+ assert db_request.session.flash.calls == [
+ pretend.call("This role no longer exists", queue="error")
+ ]
+
+ def test_delete_role_no_confirm(self, db_request):
+ project = ProjectFactory.create(name="foo")
+ user = UserFactory.create(username="bar")
+ role = RoleFactory.create(project=project, user=user)
+
+ db_request.route_path = pretend.call_recorder(lambda *a, **kw: "/the-redirect/")
+ db_request.session = pretend.stub(
+ flash=pretend.call_recorder(lambda *a, **kw: None)
+ )
+ db_request.matchdict["role_id"] = role.id
+ db_request.user = UserFactory.create()
+ db_request.remote_addr = "192.168.1.1"
+
+ with pytest.raises(HTTPSeeOther):
+ views.delete_role(project, db_request)
+
+ assert db_request.session.flash.calls == [
+ pretend.call("Confirm the request", queue="error")
+ ]
| Add admin ability to reassign project to another user
Legacy PyPI allowed admins to take over an existing project, but Warehouse doesn't have a mechanism to allow an admin to re-assign a project to another user.
| @nlhkabu I'm going to work on this during the ep18 sprints.
The plan is to add an expandable section at the bottom of the project's "view" page in the admin similar to "Delete Project" or "Set Upload Limit"
Since a project might have multiple owners, it would be ideal if this feature allowed us to add/remove roles for a given project.
(Also probably worth mentioning that the users in the dev DB with admin access are `dstufft`, `ewdurbin` and `di`) | 2018-07-29T11:46:51Z | [] | [] |
pypi/warehouse | 4,541 | pypi__warehouse-4541 | [
"4471"
] | 9b45a9132aaac2203f34b6c7979c8567d192cdfc | diff --git a/warehouse/accounts/__init__.py b/warehouse/accounts/__init__.py
--- a/warehouse/accounts/__init__.py
+++ b/warehouse/accounts/__init__.py
@@ -13,6 +13,7 @@
import datetime
from pyramid.authorization import ACLAuthorizationPolicy
+from pyramid.httpexceptions import HTTPUnauthorized
from pyramid_multiauth import MultiAuthenticationPolicy
from warehouse.accounts.interfaces import (
@@ -21,17 +22,22 @@
IPasswordBreachedService,
)
from warehouse.accounts.services import (
+ HaveIBeenPwnedPasswordBreachedService,
+ NullPasswordBreachedService,
TokenServiceFactory,
database_login_factory,
- hibp_password_breach_factory,
)
from warehouse.accounts.auth_policy import (
BasicAuthAuthenticationPolicy,
SessionAuthenticationPolicy,
)
+from warehouse.email import send_password_compromised_email
from warehouse.rate_limiting import RateLimit, IRateLimiter
+__all__ = ["NullPasswordBreachedService", "HaveIBeenPwnedPasswordBreachedService"]
+
+
REDIRECT_FIELD_NAME = "next"
@@ -45,6 +51,8 @@ def _login(username, password, request, check_password_tags=None):
def _login_via_basic_auth(username, password, request):
+ login_service = request.find_service(IUserService, context=None)
+
result = _login(
username,
password,
@@ -59,9 +67,24 @@ def _login_via_basic_auth(username, password, request):
# with this information, but for now it will provide metrics into how many
# authentications are using compromised credentials.
breach_service = request.find_service(IPasswordBreachedService, context=None)
- breach_service.check_password(
+ if breach_service.check_password(
password, tags=["method:auth", "auth_method:basic"]
- )
+ ):
+ user = login_service.get_user(login_service.find_userid(username))
+ send_password_compromised_email(request, user)
+ login_service.disable_password(user.id)
+
+ # This technically violates the contract a little bit, this function is
+ # meant to return None if the user cannot log in. However we want to present
+ # a different error message than is normal when we're denying the log in
+ # becasue of a compromised password. So to do that, we'll need to raise a
+ # HTTPError that'll ultimately get returned to the client. This is OK to do
+ # here because we've already successfully authenticated the credentials, so
+ # it won't screw up the fall through to other authentication mechanisms
+ # (since we wouldn't have fell through to them anyways).
+ resp = HTTPUnauthorized()
+ resp.status = f"{resp.status_code} {breach_service.failure_message_plain}"
+ raise resp
return result
@@ -104,8 +127,13 @@ def includeme(config):
)
# Register our password breach detection service.
+ breached_pw_class = config.maybe_dotted(
+ config.registry.settings.get(
+ "breached_passwords.backend", HaveIBeenPwnedPasswordBreachedService
+ )
+ )
config.register_service_factory(
- hibp_password_breach_factory, IPasswordBreachedService
+ breached_pw_class.create_service, IPasswordBreachedService
)
# Register our authentication and authorization policies
diff --git a/warehouse/accounts/forms.py b/warehouse/accounts/forms.py
--- a/warehouse/accounts/forms.py
+++ b/warehouse/accounts/forms.py
@@ -17,6 +17,7 @@
from warehouse import forms
from warehouse.accounts.interfaces import TooManyFailedLogins
+from warehouse.email import send_password_compromised_email
class UsernameMixin:
@@ -180,9 +181,29 @@ def __init__(self, *args, user_service, **kwargs):
class LoginForm(PasswordMixin, UsernameMixin, forms.Form):
- def __init__(self, *args, user_service, **kwargs):
+ def __init__(self, *args, request, user_service, breach_service, **kwargs):
super().__init__(*args, **kwargs)
+ self.request = request
self.user_service = user_service
+ self.breach_service = breach_service
+
+ def validate_password(self, field):
+ super().validate_password(field)
+
+ # If we have a user ID, then we'll go and check it against our breached password
+ # service. If the password has appeared in a breach or is otherwise compromised
+ # we will disable the user and reject the login.
+ userid = self.user_service.find_userid(self.username.data)
+ if userid is not None:
+ if self.breach_service.check_password(
+ field.data, tags=["method:auth", "auth_method:login_form"]
+ ):
+ user = self.user_service.get_user(userid)
+ send_password_compromised_email(self.request, user)
+ self.user_service.disable_password(user.id)
+ raise wtforms.validators.ValidationError(
+ jinja2.Markup(self.breach_service.failure_message)
+ )
class RequestPasswordResetForm(forms.Form):
diff --git a/warehouse/accounts/interfaces.py b/warehouse/accounts/interfaces.py
--- a/warehouse/accounts/interfaces.py
+++ b/warehouse/accounts/interfaces.py
@@ -86,6 +86,12 @@ def update_user(user_id, **changes):
Updates the user object
"""
+ def disable_password(user_id):
+ """
+ Disables the given user's password, preventing further login until the user
+ resets their password.
+ """
+
class ITokenService(Interface):
def dumps(data):
@@ -101,6 +107,9 @@ def loads(token):
class IPasswordBreachedService(Interface):
failure_message = Attribute("The message to describe the failure that occured")
+ failure_message_plain = Attribute(
+ "The message to describe the failure that occured in plain text"
+ )
def check_password(password, *, tags=None):
"""
diff --git a/warehouse/accounts/services.py b/warehouse/accounts/services.py
--- a/warehouse/accounts/services.py
+++ b/warehouse/accounts/services.py
@@ -221,6 +221,10 @@ def update_user(self, user_id, **changes):
setattr(user, attr, value)
return user
+ def disable_password(self, user_id):
+ user = self.get_user(user_id)
+ user.password = self.hasher.disable()
+
@implementer(ITokenService)
class TokenService:
@@ -282,6 +286,11 @@ def __eq__(self, other):
@implementer(IPasswordBreachedService)
class HaveIBeenPwnedPasswordBreachedService:
+
+ _failure_message_preamble = (
+ "This password appears in a breach or has been compromised and cannot be used."
+ )
+
def __init__(
self,
*,
@@ -295,11 +304,29 @@ def __init__(
self._metrics = metrics
self._help_url = help_url
+ @classmethod
+ def create_service(cls, context, request):
+ return cls(
+ session=request.http,
+ metrics=request.find_service(IMetricsService, context=None),
+ help_url=request.help_url(_anchor="compromised-password"),
+ )
+
@property
def failure_message(self):
- message = "This password has appeared in a breach or has otherwise been compromised and cannot be used."
+ message = self._failure_message_preamble
if self._help_url:
- message += f' See <a href="{self._help_url}">this FAQ entry</a> for more information.'
+ message += (
+ f' See <a href="{self._help_url}">this FAQ entry</a> for more '
+ "information."
+ )
+ return message
+
+ @property
+ def failure_message_plain(self):
+ message = self._failure_message_preamble
+ if self._help_url:
+ message += f" See the FAQ entry at {self._help_url} for more information."
return message
def _metrics_increment(self, *args, **kwargs):
@@ -363,9 +390,16 @@ def check_password(self, password, *, tags=None):
return False
-def hibp_password_breach_factory(context, request):
- return HaveIBeenPwnedPasswordBreachedService(
- session=request.http,
- metrics=request.find_service(IMetricsService, context=None),
- help_url=request.help_url(_anchor="compromised-password"),
- )
+@implementer(IPasswordBreachedService)
+class NullPasswordBreachedService:
+ failure_message = "This password appears in a breach."
+ failure_message_plain = "This password appears in a breach."
+
+ @classmethod
+ def create_service(cls, context, request):
+ return cls()
+
+ def check_password(self, password, *, tags=None):
+ # This service allows *every* password as a non-breached password. It will never
+ # tell a user their password isn't good enough.
+ return False
diff --git a/warehouse/accounts/views.py b/warehouse/accounts/views.py
--- a/warehouse/accounts/views.py
+++ b/warehouse/accounts/views.py
@@ -110,7 +110,9 @@ def login(request, redirect_field_name=REDIRECT_FIELD_NAME, _form_class=LoginFor
form = _form_class(
request.POST,
+ request=request,
user_service=user_service,
+ breach_service=breach_service,
check_password_metrics_tags=["method:auth", "auth_method:login_form"],
)
@@ -120,13 +122,6 @@ def login(request, redirect_field_name=REDIRECT_FIELD_NAME, _form_class=LoginFor
username = form.username.data
userid = user_service.find_userid(username)
- # Run our password through our breach validation. We don't currently do
- # anything with this information, but for now it will provide metrics into
- # how many authentications are using compromised credentials.
- breach_service.check_password(
- form.password.data, tags=["method:auth", "auth_method:login_form"]
- )
-
# If the user-originating redirection url is not safe, then
# redirect to the index instead.
if not redirect_to or not is_safe_url(url=redirect_to, host=request.host):
diff --git a/warehouse/config.py b/warehouse/config.py
--- a/warehouse/config.py
+++ b/warehouse/config.py
@@ -220,6 +220,7 @@ def configure(settings=None):
maybe_set_compound(settings, "origin_cache", "backend", "ORIGIN_CACHE")
maybe_set_compound(settings, "mail", "backend", "MAIL_BACKEND")
maybe_set_compound(settings, "metrics", "backend", "METRICS_BACKEND")
+ maybe_set_compound(settings, "breached_passwords", "backend", "BREACHED_PASSWORDS")
# Add the settings we use when the environment is set to development.
if settings["warehouse.env"] == Environment.development:
diff --git a/warehouse/email/__init__.py b/warehouse/email/__init__.py
--- a/warehouse/email/__init__.py
+++ b/warehouse/email/__init__.py
@@ -158,6 +158,11 @@ def send_password_change_email(request, user):
return {"username": user.username}
+@_email("password-compromised", allow_unverified=True)
+def send_password_compromised_email(request, user):
+ return {}
+
+
@_email("account-deleted")
def send_account_deletion_email(request, user):
return {"username": user.username}
| diff --git a/tests/unit/accounts/test_core.py b/tests/unit/accounts/test_core.py
--- a/tests/unit/accounts/test_core.py
+++ b/tests/unit/accounts/test_core.py
@@ -16,6 +16,8 @@
import pretend
import pytest
+from pyramid.httpexceptions import HTTPUnauthorized
+
from warehouse import accounts
from warehouse.accounts.interfaces import (
IUserService,
@@ -24,8 +26,8 @@
)
from warehouse.accounts.services import (
TokenServiceFactory,
+ HaveIBeenPwnedPasswordBreachedService,
database_login_factory,
- hibp_password_breach_factory,
)
from warehouse.rate_limiting import RateLimit, IRateLimiter
@@ -93,13 +95,16 @@ def test_with_valid_password(self, monkeypatch):
assert service.update_user.calls == [pretend.call(userid, last_login=now)]
assert authenticate.calls == [pretend.call(userid, request)]
- def test_via_basic_auth_no_user(self, monkeypatch):
+ def test_via_basic_auth_no_user(self, monkeypatch, pyramid_services):
login = pretend.call_recorder(lambda *a, **kw: None)
monkeypatch.setattr(accounts, "_login", login)
+ login_service = pretend.stub()
+ pyramid_services.register_service(IUserService, None, login_service)
+
username = pretend.stub()
password = pretend.stub()
- request = pretend.stub()
+ request = pretend.stub(find_service=pyramid_services.find_service)
assert accounts._login_via_basic_auth(username, password, request) is None
assert login.calls == [
@@ -111,17 +116,23 @@ def test_via_basic_auth_no_user(self, monkeypatch):
)
]
- def test_via_basic_auth_with_user(self, monkeypatch):
+ def test_via_basic_auth_with_user(self, monkeypatch, pyramid_services):
login = pretend.call_recorder(lambda *a, **kw: ["foo"])
monkeypatch.setattr(accounts, "_login", login)
+ user_service = pretend.stub()
breach_service = pretend.stub(
check_password=pretend.call_recorder(lambda pw, tags=None: False)
)
+ pyramid_services.register_service(IUserService, None, user_service)
+ pyramid_services.register_service(
+ IPasswordBreachedService, None, breach_service
+ )
+
username = pretend.stub()
password = pretend.stub()
- request = pretend.stub(find_service=lambda iface, context: breach_service)
+ request = pretend.stub(find_service=pyramid_services.find_service)
assert accounts._login_via_basic_auth(username, password, request) == ["foo"]
assert login.calls == [
@@ -136,6 +147,52 @@ def test_via_basic_auth_with_user(self, monkeypatch):
pretend.call(password, tags=["method:auth", "auth_method:basic"])
]
+ def test_via_basic_auth_compromised(self, monkeypatch, pyramid_services):
+ login = pretend.call_recorder(lambda *a, **kw: ["foo"])
+ monkeypatch.setattr(accounts, "_login", login)
+
+ send_email = pretend.call_recorder(lambda *a, **kw: None)
+ monkeypatch.setattr(accounts, "send_password_compromised_email", send_email)
+
+ user = pretend.stub(id=1)
+
+ user_service = pretend.stub(
+ find_userid=lambda username: 1,
+ get_user=lambda user_id: user,
+ disable_password=pretend.call_recorder(lambda user_id: None),
+ )
+ breach_service = pretend.stub(
+ failure_message_plain="Bad Password!",
+ check_password=pretend.call_recorder(lambda pw, tags=None: True),
+ )
+
+ pyramid_services.register_service(IUserService, None, user_service)
+ pyramid_services.register_service(
+ IPasswordBreachedService, None, breach_service
+ )
+
+ username = pretend.stub()
+ password = pretend.stub()
+ request = pretend.stub(find_service=pyramid_services.find_service)
+
+ with pytest.raises(HTTPUnauthorized) as excinfo:
+ accounts._login_via_basic_auth(username, password, request)
+
+ assert excinfo.value.status == "401 Bad Password!"
+ assert login.calls == [
+ pretend.call(
+ username,
+ password,
+ request,
+ check_password_tags=["method:auth", "auth_method:basic"],
+ )
+ ]
+ assert breach_service.check_password.calls == [
+ pretend.call(password, tags=["method:auth", "auth_method:basic"])
+ ]
+ assert user_service.disable_password.calls == [pretend.call(1)]
+ assert send_email.calls == [pretend.call(request, user)]
+
class TestAuthenticate:
@pytest.mark.parametrize(
@@ -199,12 +256,14 @@ def test_includeme(monkeypatch):
monkeypatch.setattr(accounts, "ACLAuthorizationPolicy", authz_cls)
config = pretend.stub(
+ registry=pretend.stub(settings={}),
register_service_factory=pretend.call_recorder(
lambda factory, iface, name=None: None
),
add_request_method=pretend.call_recorder(lambda f, name, reify: None),
set_authentication_policy=pretend.call_recorder(lambda p: None),
set_authorization_policy=pretend.call_recorder(lambda p: None),
+ maybe_dotted=pretend.call_recorder(lambda path: path),
)
accounts.includeme(config)
@@ -215,7 +274,10 @@ def test_includeme(monkeypatch):
TokenServiceFactory(name="password"), ITokenService, name="password"
),
pretend.call(TokenServiceFactory(name="email"), ITokenService, name="email"),
- pretend.call(hibp_password_breach_factory, IPasswordBreachedService),
+ pretend.call(
+ HaveIBeenPwnedPasswordBreachedService.create_service,
+ IPasswordBreachedService,
+ ),
pretend.call(RateLimit("10 per 5 minutes"), IRateLimiter, name="user.login"),
pretend.call(
RateLimit("1000 per 5 minutes"), IRateLimiter, name="global.login"
diff --git a/tests/unit/accounts/test_forms.py b/tests/unit/accounts/test_forms.py
--- a/tests/unit/accounts/test_forms.py
+++ b/tests/unit/accounts/test_forms.py
@@ -20,16 +20,26 @@
class TestLoginForm:
def test_creation(self):
+ request = pretend.stub()
user_service = pretend.stub()
- form = forms.LoginForm(user_service=user_service)
+ breach_service = pretend.stub()
+ form = forms.LoginForm(
+ request=request, user_service=user_service, breach_service=breach_service
+ )
+ assert form.request is request
assert form.user_service is user_service
+ assert form.breach_service is breach_service
def test_validate_username_with_no_user(self):
+ request = pretend.stub()
user_service = pretend.stub(
find_userid=pretend.call_recorder(lambda userid: None)
)
- form = forms.LoginForm(user_service=user_service)
+ breach_service = pretend.stub()
+ form = forms.LoginForm(
+ request=request, user_service=user_service, breach_service=breach_service
+ )
field = pretend.stub(data="my_username")
with pytest.raises(wtforms.validators.ValidationError):
@@ -38,8 +48,12 @@ def test_validate_username_with_no_user(self):
assert user_service.find_userid.calls == [pretend.call("my_username")]
def test_validate_username_with_user(self):
+ request = pretend.stub()
user_service = pretend.stub(find_userid=pretend.call_recorder(lambda userid: 1))
- form = forms.LoginForm(user_service=user_service)
+ breach_service = pretend.stub()
+ form = forms.LoginForm(
+ request=request, user_service=user_service, breach_service=breach_service
+ )
field = pretend.stub(data="my_username")
form.validate_username(field)
@@ -47,48 +61,73 @@ def test_validate_username_with_user(self):
assert user_service.find_userid.calls == [pretend.call("my_username")]
def test_validate_password_no_user(self):
+ request = pretend.stub()
user_service = pretend.stub(
find_userid=pretend.call_recorder(lambda userid: None)
)
+ breach_service = pretend.stub()
form = forms.LoginForm(
- data={"username": "my_username"}, user_service=user_service
+ data={"username": "my_username"},
+ request=request,
+ user_service=user_service,
+ breach_service=breach_service,
)
field = pretend.stub(data="password")
form.validate_password(field)
- assert user_service.find_userid.calls == [pretend.call("my_username")]
+ assert user_service.find_userid.calls == [
+ pretend.call("my_username"),
+ pretend.call("my_username"),
+ ]
def test_validate_password_ok(self):
+ request = pretend.stub()
user_service = pretend.stub(
find_userid=pretend.call_recorder(lambda userid: 1),
check_password=pretend.call_recorder(
lambda userid, password, tags=None: True
),
)
+ breach_service = pretend.stub(
+ check_password=pretend.call_recorder(lambda pw, tags: False)
+ )
form = forms.LoginForm(
data={"username": "my_username"},
+ request=request,
user_service=user_service,
+ breach_service=breach_service,
check_password_metrics_tags=["bar"],
)
field = pretend.stub(data="pw")
form.validate_password(field)
- assert user_service.find_userid.calls == [pretend.call("my_username")]
+ assert user_service.find_userid.calls == [
+ pretend.call("my_username"),
+ pretend.call("my_username"),
+ ]
assert user_service.check_password.calls == [
pretend.call(1, "pw", tags=["bar"])
]
+ assert breach_service.check_password.calls == [
+ pretend.call("pw", tags=["method:auth", "auth_method:login_form"])
+ ]
def test_validate_password_notok(self, db_session):
+ request = pretend.stub()
user_service = pretend.stub(
find_userid=pretend.call_recorder(lambda userid: 1),
check_password=pretend.call_recorder(
lambda userid, password, tags=None: False
),
)
+ breach_service = pretend.stub()
form = forms.LoginForm(
- data={"username": "my_username"}, user_service=user_service
+ data={"username": "my_username"},
+ request=request,
+ user_service=user_service,
+ breach_service=breach_service,
)
field = pretend.stub(data="pw")
@@ -103,12 +142,17 @@ def test_validate_password_too_many_failed(self):
def check_password(userid, password, tags=None):
raise TooManyFailedLogins(resets_in=None)
+ request = pretend.stub()
user_service = pretend.stub(
find_userid=pretend.call_recorder(lambda userid: 1),
check_password=check_password,
)
+ breach_service = pretend.stub()
form = forms.LoginForm(
- data={"username": "my_username"}, user_service=user_service
+ data={"username": "my_username"},
+ request=request,
+ user_service=user_service,
+ breach_service=breach_service,
)
field = pretend.stub(data="pw")
@@ -118,6 +162,33 @@ def check_password(userid, password, tags=None):
assert user_service.find_userid.calls == [pretend.call("my_username")]
assert user_service.check_password.calls == [pretend.call(1, "pw", tags=None)]
+ def test_password_breached(self, monkeypatch):
+ send_email = pretend.call_recorder(lambda *a, **kw: None)
+ monkeypatch.setattr(forms, "send_password_compromised_email", send_email)
+
+ user = pretend.stub(id=1)
+ request = pretend.stub()
+ user_service = pretend.stub(
+ find_userid=lambda _: 1,
+ get_user=lambda _: user,
+ check_password=lambda userid, pw, tags=None: True,
+ disable_password=pretend.call_recorder(lambda user_id: None),
+ )
+ breach_service = pretend.stub(
+ check_password=lambda pw, tags=None: True, failure_message="Bad Password!"
+ )
+
+ form = forms.LoginForm(
+ data={"password": "password"},
+ request=request,
+ user_service=user_service,
+ breach_service=breach_service,
+ )
+ assert not form.validate()
+ assert form.password.errors.pop() == "Bad Password!"
+ assert user_service.disable_password.calls == [pretend.call(1)]
+ assert send_email.calls == [pretend.call(request, user)]
+
class TestRegistrationForm:
def test_create(self):
diff --git a/tests/unit/accounts/test_services.py b/tests/unit/accounts/test_services.py
--- a/tests/unit/accounts/test_services.py
+++ b/tests/unit/accounts/test_services.py
@@ -25,6 +25,7 @@
from warehouse.accounts.interfaces import (
IUserService,
ITokenService,
+ IPasswordBreachedService,
TokenExpired,
TokenInvalid,
TokenMissing,
@@ -299,6 +300,17 @@ def test_get_user_by_email_failure(self, user_service):
assert found_user is None
+ def test_disable_password(self, user_service):
+ user = UserFactory.create()
+
+ # Need to give the user a good password first.
+ user_service.update_user(user.id, password="foo")
+ assert user.password != "!"
+
+ # Now we'll actually test our disable function.
+ user_service.disable_password(user.id)
+ assert user.password == "!"
+
class TestTokenService:
def test_verify_service(self):
@@ -443,6 +455,11 @@ def test_token_service_factory_eq():
class TestHaveIBeenPwnedPasswordBreachedService:
+ def test_verify_service(self):
+ assert verifyClass(
+ IPasswordBreachedService, services.HaveIBeenPwnedPasswordBreachedService
+ )
+
@pytest.mark.parametrize(
("password", "prefix", "expected", "dataset"),
[
@@ -548,7 +565,9 @@ def test_factory(self):
}[(iface, context)],
help_url=lambda _anchor=None: f"http://localhost/help/#{_anchor}",
)
- svc = services.hibp_password_breach_factory(context, request)
+ svc = services.HaveIBeenPwnedPasswordBreachedService.create_service(
+ context, request
+ )
assert svc._http is request.http
assert isinstance(svc._metrics, NullMetrics)
@@ -560,15 +579,15 @@ def test_factory(self):
(
None,
(
- "This password has appeared in a breach or has otherwise "
- "been compromised and cannot be used."
+ "This password appears in a breach or has been compromised and "
+ "cannot be used."
),
),
(
"http://localhost/help/#compromised-password",
(
- "This password has appeared in a breach or has otherwise "
- "been compromised and cannot be used. See "
+ "This password appears in a breach or has been compromised and "
+ "cannot be used. See "
'<a href="http://localhost/help/#compromised-password">'
"this FAQ entry</a> for more information."
),
@@ -584,5 +603,60 @@ def test_failure_message(self, help_url, expected):
}[(iface, context)],
help_url=lambda _anchor=None: help_url,
)
- svc = services.hibp_password_breach_factory(context, request)
+ svc = services.HaveIBeenPwnedPasswordBreachedService.create_service(
+ context, request
+ )
assert svc.failure_message == expected
+
+ @pytest.mark.parametrize(
+ ("help_url", "expected"),
+ [
+ (
+ None,
+ (
+ "This password appears in a breach or has been compromised and "
+ "cannot be used."
+ ),
+ ),
+ (
+ "http://localhost/help/#compromised-password",
+ (
+ "This password appears in a breach or has been compromised and "
+ "cannot be used. See the FAQ entry at "
+ "http://localhost/help/#compromised-password for more information."
+ ),
+ ),
+ ],
+ )
+ def test_failure_message_plain(self, help_url, expected):
+ context = pretend.stub()
+ request = pretend.stub(
+ http=pretend.stub(),
+ find_service=lambda iface, context: {
+ (IMetricsService, None): NullMetrics()
+ }[(iface, context)],
+ help_url=lambda _anchor=None: help_url,
+ )
+ svc = services.HaveIBeenPwnedPasswordBreachedService.create_service(
+ context, request
+ )
+ assert svc.failure_message_plain == expected
+
+
+class TestNullPasswordBreachedService:
+ def test_verify_service(self):
+ assert verifyClass(
+ IPasswordBreachedService, services.NullPasswordBreachedService
+ )
+
+ def test_check_password(self):
+ svc = services.NullPasswordBreachedService()
+ assert not svc.check_password("password")
+
+ def test_factory(self):
+ context = pretend.stub()
+ request = pretend.stub()
+ svc = services.NullPasswordBreachedService.create_service(context, request)
+
+ assert isinstance(svc, services.NullPasswordBreachedService)
+ assert not svc.check_password("hunter2")
diff --git a/tests/unit/accounts/test_views.py b/tests/unit/accounts/test_views.py
--- a/tests/unit/accounts/test_views.py
+++ b/tests/unit/accounts/test_views.py
@@ -87,9 +87,7 @@ def test_get_returns_form(self, pyramid_request, pyramid_services, next_url):
)
form_obj = pretend.stub()
- form_class = pretend.call_recorder(
- lambda d, user_service, check_password_metrics_tags: form_obj
- )
+ form_class = pretend.call_recorder(lambda d, **kw: form_obj)
if next_url is not None:
pyramid_request.GET["next"] = next_url
@@ -103,7 +101,9 @@ def test_get_returns_form(self, pyramid_request, pyramid_services, next_url):
assert form_class.calls == [
pretend.call(
pyramid_request.POST,
+ request=pyramid_request,
user_service=user_service,
+ breach_service=breach_service,
check_password_metrics_tags=["method:auth", "auth_method:login_form"],
)
]
@@ -124,9 +124,7 @@ def test_post_invalid_returns_form(
if next_url is not None:
pyramid_request.POST["next"] = next_url
form_obj = pretend.stub(validate=pretend.call_recorder(lambda: False))
- form_class = pretend.call_recorder(
- lambda d, user_service, check_password_metrics_tags: form_obj
- )
+ form_class = pretend.call_recorder(lambda d, **kw: form_obj)
result = views.login(pyramid_request, _form_class=form_class)
assert metrics.increment.calls == []
@@ -138,7 +136,9 @@ def test_post_invalid_returns_form(
assert form_class.calls == [
pretend.call(
pyramid_request.POST,
+ request=pyramid_request,
user_service=user_service,
+ breach_service=breach_service,
check_password_metrics_tags=["method:auth", "auth_method:login_form"],
)
]
@@ -183,9 +183,7 @@ def test_post_validate_redirects(
username=pretend.stub(data="theuser"),
password=pretend.stub(data="password"),
)
- form_class = pretend.call_recorder(
- lambda d, user_service, check_password_metrics_tags: form_obj
- )
+ form_class = pretend.call_recorder(lambda d, **kw: form_obj)
pyramid_request.route_path = pretend.call_recorder(lambda a: "/the-redirect")
@@ -204,7 +202,9 @@ def test_post_validate_redirects(
assert form_class.calls == [
pretend.call(
pyramid_request.POST,
+ request=pyramid_request,
user_service=user_service,
+ breach_service=breach_service,
check_password_metrics_tags=["method:auth", "auth_method:login_form"],
)
]
@@ -250,9 +250,7 @@ def test_post_validate_no_redirects(
username=pretend.stub(data="theuser"),
password=pretend.stub(data="password"),
)
- form_class = pretend.call_recorder(
- lambda d, user_service, check_password_metrics_tags: form_obj
- )
+ form_class = pretend.call_recorder(lambda d, **kw: form_obj)
pyramid_request.route_path = pretend.call_recorder(lambda a: "/the-redirect")
result = views.login(pyramid_request, _form_class=form_class)
diff --git a/tests/unit/email/test_init.py b/tests/unit/email/test_init.py
--- a/tests/unit/email/test_init.py
+++ b/tests/unit/email/test_init.py
@@ -474,6 +474,57 @@ def test_password_change_email_unverified(
assert send_email.delay.calls == []
+class TestPasswordCompromisedEmail:
+ @pytest.mark.parametrize("verified", [True, False])
+ def test_password_compromised_email(
+ self, pyramid_request, pyramid_config, monkeypatch, verified
+ ):
+ stub_user = pretend.stub(
+ username="username",
+ name="",
+ email="[email protected]",
+ primary_email=pretend.stub(email="[email protected]", verified=verified),
+ )
+ subject_renderer = pyramid_config.testing_add_renderer(
+ "email/password-compromised/subject.txt"
+ )
+ subject_renderer.string_response = "Email Subject"
+ body_renderer = pyramid_config.testing_add_renderer(
+ "email/password-compromised/body.txt"
+ )
+ body_renderer.string_response = "Email Body"
+ html_renderer = pyramid_config.testing_add_renderer(
+ "email/password-compromised/body.html"
+ )
+ html_renderer.string_response = "Email HTML Body"
+
+ send_email = pretend.stub(
+ delay=pretend.call_recorder(lambda *args, **kwargs: None)
+ )
+ pyramid_request.task = pretend.call_recorder(lambda *args, **kwargs: send_email)
+ monkeypatch.setattr(email, "send_email", send_email)
+
+ result = email.send_password_compromised_email(pyramid_request, stub_user)
+
+ assert result == {}
+ assert pyramid_request.task.calls == [pretend.call(send_email)]
+ assert send_email.delay.calls == [
+ pretend.call(
+ f"{stub_user.username} <{stub_user.email}>",
+ attr.asdict(
+ EmailMessage(
+ subject="Email Subject",
+ body_text="Email Body",
+ body_html=(
+ "<html>\n<head></head>\n"
+ "<body><p>Email HTML Body</p></body>\n</html>\n"
+ ),
+ )
+ ),
+ )
+ ]
+
+
class TestAccountDeletionEmail:
def test_account_deletion_email(self, pyramid_request, pyramid_config, monkeypatch):
| Notify & restrict users with breached/compromised passwords
As a follow up to https://github.com/pypa/warehouse/pull/4468, we should figure out the best way to get people who have an *existing* password that has been breached/compromised to change their password to something that hasn't been reused. This is somewhat complicated by the fact that we (rightfully) cannot access the plaintext password *except* when the user is logging into the site.
A few ideas for what we could possibly do here:
* Just check on user login and send a flash message asking them to change their password.
* Causing an error to occur in the basic authentication scheme whenever a user tries to login with breached credentials.
* This would effectively block uploads until the user changes their password to a good one.
* Trigger an email anytime the user authenticates with breached credentials, telling them their credentials have been breached, and asking them to change them.
* Flag the user account as having a compromised/breached password, and display a persistent warning in the UI (similar to unverified email).
* One potential issue with this is that it leaks additional persistent data about the user's password into the database. If a database gets leaked, an attacker would have a list of users to focus on to try and attempt to break because they have passwords that likely exist in their password dictionary.
* Store a flag in the user's session indicating they have a compromised/breached password, and prevent accessing any other features until they rectify this situation.
* This has similar problems to storing a flag in the database, except the storage is time limited to how long the user's session can live (I think 12 hours?), which drastically decreases the potential risk. It also lives in a separate system (currently, though there is some desire to move sessions into the database) so it would require compromising two systems instead of one.
One thing to keep in mind, is that for a lot of users, they very rarely log into the UI, and their primary interaction is using an upload client like ``twine`` so a strategy that relies entirely on signals in the UI is not likely to reach a large portion of users. Another thing to keep in mind is that in the future, we are almost certainly going to move to some form of an API key (https://github.com/pypa/warehouse/issues/994).
Given that, I think the right answer here is likely:
- Store a flag in the user's session (or perhaps the DB?) and prevent them from doing anything else in the UI, until they've changed their password.
- If we have a flag stored in the database, maybe we want to periodically send out an email telling them to change their password? This could get spammy though.
- Block basic authentication when a compromised password is being used.
- This effectively blocks uploads for now, however in the future when we add API keys, if someone is using an API key, it will *not* block their authentication then.
- If we're storing the flag for the first item in the DB, then trying to authenticate with a compromised password in the database should also set that flag.
This means that immediately, having a compromised password more or less blocks your ability to do anything until you change it. However once we get API keys, you'll still be able to upload if your account has a compromised password (since presumably the API key isn't also compromised). If an attacker finds a compromised password and logs into the user's account with it, they'll be forced to change the password before they can use the account for anything else, which will trigger an email to the primary address on file indicating that the password has been changed. If they didn't change their password they'll ideally freak out appropriately and start reseting passwords and contacting us.
| I was doing some research on this, and it looks like Amazon might preemptively look for people's login credentials in password breaches, and will not only block the user from accessing the site, but will disable their account until they go through the account reset flow [source](https://twitter.com/paulpitchford/status/845310800713699328/photo/1).
I'm +1 for the active approach of simply failing authentication when a compromised password is used.
As far as rolling this out, It might make sense to begin with maintainers of projects in top N of download counts then move to 10\*N, 100\*N, 1000\*N, etc... as we get feedback on the impact and user experience of having your upload fail for a new/surprising reason.
Maintainers of well known and heavily used packages are the most likely to take action and understand the importance. Rolling this out in stages improves the security of the broader ecosystem immediately and offers us a chance to work out the snags before enforcing for all users of PyPI.
Ok new idea. Whenever someone authenticates with compromised credentials, we fail the authentication with an appropriate error message, at the same time we invalidate the password on the account and require users to reset their password.
Last night I was doing a fair amount of research on this topic, and I've been thinking about it, and I think the above is the correct way to go, and it's not super unusual. As I mentioned previously, Amazon is currently invalidating passwords on accounts with compromised credentials, and I've looked into what a number of other people are doing:
* [Eve Online](https://twitter.com/stebets/status/989907333441605632) sends an email to warn users.
* [Kogan](https://www.kogan.com/au/password-safety/) blocks creating new passwords, does not (currently?) do anything for existing accounts.
* [Bittylicious](https://blog.bittylicious.com/2018/02/have-i-been-pwned/) blocks creating new passwords, prevents certain classes of users from logging in if their passwords is compromised.
I've mentioned this to @ewdurbin, but I feel like limiting by top N packages doesn't exactly give us the segmentation we're looking for. Because we already have a natural segmentation happening in that we're only going to be able to run the check on users who are currently authenticating to PyPI.
FTR, the number of users who have logged into the site in the past 24 hours is 1501, so in a single day period of time during one of our most active days, the total possible number of users affected was ~1.5k. Our metrics on how many *users* are authenticating, but rather how many total authentications are happening. That is currently at 798 (which doesn't quite cover 24 hours).
Because we're only tracking authentications, we have to try and make some guesses here. However, we can see that ~71 of them were from users logging in via the website. Since it's unlikely that people are logging in 2-3+ times in the web in a 24 hour period of time (logging in isn't very useful general). I'd say we can say that would be ~70 affected users. Next if we look at basic auth, we can see 727 affected authentications. Trying to look at the distribution of users who logged in since then, and how many times they logged in, I'm going to guess there is another 60-70 users affected. For a total of roughly ~140 affected users in the last 24h.
Given that. I feel like a sample of ~140 users/day isn't the worst possible outcome here and is vastly simpler to add. | 2018-08-12T00:07:53Z | [] | [] |
pypi/warehouse | 4,583 | pypi__warehouse-4583 | [
"4545"
] | 0faadf067cd7db45d439870f06fbdeae1c59a623 | diff --git a/warehouse/accounts/views.py b/warehouse/accounts/views.py
--- a/warehouse/accounts/views.py
+++ b/warehouse/accounts/views.py
@@ -14,6 +14,7 @@
import hashlib
import uuid
+from first import first
from pyramid.httpexceptions import (
HTTPMovedPermanently,
HTTPSeeOther,
@@ -272,10 +273,14 @@ def request_password_reset(request, _form_class=RequestPasswordResetForm):
form = _form_class(request.POST, user_service=user_service)
if request.method == "POST" and form.validate():
user = user_service.get_user_by_username(form.username_or_email.data)
+ email = None
if user is None:
user = user_service.get_user_by_email(form.username_or_email.data)
+ email = first(
+ user.emails, key=lambda e: e.email == form.username_or_email.data
+ )
- send_password_reset_email(request, user)
+ send_password_reset_email(request, (user, email))
token_service = request.find_service(ITokenService, name="password")
n_hours = token_service.max_age // 60 // 60
diff --git a/warehouse/email/__init__.py b/warehouse/email/__init__.py
--- a/warehouse/email/__init__.py
+++ b/warehouse/email/__init__.py
@@ -122,7 +122,8 @@ def wrapper(request, user_or_users, **kwargs):
@_email("password-reset", allow_unverified=True)
-def send_password_reset_email(request, user):
+def send_password_reset_email(request, user_and_email):
+ user, _ = user_and_email
token_service = request.find_service(ITokenService, name="password")
token = token_service.dumps(
{
| diff --git a/tests/unit/accounts/test_views.py b/tests/unit/accounts/test_views.py
--- a/tests/unit/accounts/test_views.py
+++ b/tests/unit/accounts/test_views.py
@@ -506,14 +506,16 @@ def test_request_password_reset(
pretend.call(pyramid_request.POST, user_service=user_service)
]
assert send_password_reset_email.calls == [
- pretend.call(pyramid_request, stub_user)
+ pretend.call(pyramid_request, (stub_user, None))
]
def test_request_password_reset_with_email(
self, monkeypatch, pyramid_request, pyramid_config, user_service, token_service
):
- stub_user = pretend.stub(email=pretend.stub())
+ stub_user = pretend.stub(
+ email="[email protected]", emails=[pretend.stub(email="[email protected]")]
+ )
pyramid_request.method = "POST"
token_service.dumps = pretend.call_recorder(lambda a: "TOK")
user_service.get_user_by_username = pretend.call_recorder(lambda a: None)
@@ -553,18 +555,24 @@ def test_request_password_reset_with_email(
pretend.call(pyramid_request.POST, user_service=user_service)
]
assert send_password_reset_email.calls == [
- pretend.call(pyramid_request, stub_user)
+ pretend.call(pyramid_request, (stub_user, stub_user.emails[0]))
]
- def test_request_password_reset_with_wrong_credentials(
+ def test_request_password_reset_with_non_primary_email(
self, monkeypatch, pyramid_request, pyramid_config, user_service, token_service
):
- stub_user = pretend.stub(username=pretend.stub())
+ stub_user = pretend.stub(
+ email="[email protected]",
+ emails=[
+ pretend.stub(email="[email protected]"),
+ pretend.stub(email="[email protected]"),
+ ],
+ )
pyramid_request.method = "POST"
token_service.dumps = pretend.call_recorder(lambda a: "TOK")
user_service.get_user_by_username = pretend.call_recorder(lambda a: None)
- user_service.get_user_by_email = pretend.call_recorder(lambda a: None)
+ user_service.get_user_by_email = pretend.call_recorder(lambda a: stub_user)
pyramid_request.find_service = pretend.call_recorder(
lambda interface, **kw: {
IUserService: user_service,
@@ -572,7 +580,7 @@ def test_request_password_reset_with_wrong_credentials(
}[interface]
)
form_obj = pretend.stub(
- username_or_email=pretend.stub(data=stub_user.username),
+ username_or_email=pretend.stub(data="[email protected]"),
validate=pretend.call_recorder(lambda: True),
)
form_class = pretend.call_recorder(lambda d, user_service: form_obj)
@@ -588,10 +596,10 @@ def test_request_password_reset_with_wrong_credentials(
assert result == {"n_hours": n_hours}
assert user_service.get_user_by_username.calls == [
- pretend.call(stub_user.username)
+ pretend.call("[email protected]")
]
assert user_service.get_user_by_email.calls == [
- pretend.call(stub_user.username)
+ pretend.call("[email protected]")
]
assert pyramid_request.find_service.calls == [
pretend.call(IUserService, context=None),
@@ -601,7 +609,9 @@ def test_request_password_reset_with_wrong_credentials(
assert form_class.calls == [
pretend.call(pyramid_request.POST, user_service=user_service)
]
- assert send_password_reset_email.calls == [pretend.call(pyramid_request, None)]
+ assert send_password_reset_email.calls == [
+ pretend.call(pyramid_request, (stub_user, stub_user.emails[1]))
+ ]
def test_redirect_authenticated_user(self):
pyramid_request = pretend.stub(authenticated_userid=1)
diff --git a/tests/unit/email/test_init.py b/tests/unit/email/test_init.py
--- a/tests/unit/email/test_init.py
+++ b/tests/unit/email/test_init.py
@@ -235,9 +235,23 @@ def retry(exc):
class TestSendPasswordResetEmail:
- @pytest.mark.parametrize("verified", [True, False])
+ @pytest.mark.parametrize(
+ ("verified", "email_addr"),
+ [
+ (True, None),
+ (False, None),
+ (True, "[email protected]"),
+ (False, "[email protected]"),
+ ],
+ )
def test_send_password_reset_email(
- self, verified, pyramid_request, pyramid_config, token_service, monkeypatch
+ self,
+ verified,
+ email_addr,
+ pyramid_request,
+ pyramid_config,
+ token_service,
+ monkeypatch,
):
stub_user = pretend.stub(
@@ -249,6 +263,10 @@ def test_send_password_reset_email(
last_login="last_login",
password_date="password_date",
)
+ if email_addr is None:
+ stub_email = None
+ else:
+ stub_email = pretend.stub(email=email_addr, verified=verified)
pyramid_request.method = "POST"
token_service.dumps = pretend.call_recorder(lambda a: "TOKEN")
pyramid_request.find_service = pretend.call_recorder(
@@ -274,7 +292,9 @@ def test_send_password_reset_email(
pyramid_request.task = pretend.call_recorder(lambda *args, **kwargs: send_email)
monkeypatch.setattr(email, "send_email", send_email)
- result = email.send_password_reset_email(pyramid_request, stub_user)
+ result = email.send_password_reset_email(
+ pyramid_request, (stub_user, stub_email)
+ )
assert result == {
"token": "TOKEN",
@@ -300,7 +320,9 @@ def test_send_password_reset_email(
assert pyramid_request.task.calls == [pretend.call(send_email)]
assert send_email.delay.calls == [
pretend.call(
- "name_value <" + stub_user.email + ">",
+ "name_value <"
+ + (stub_user.email if email_addr is None else email_addr)
+ + ">",
attr.asdict(
EmailMessage(
subject="Email Subject",
| Send password reset to the specified email instead of Primary
Currently the password reset page allows specifying either a username or an email address to reset your password for. However, if you supply an email address that isn't the primary email address on the account, it will successfully find the account, but will send the password reset to the primary address anyways. This negates a lot of the benefit of having secondary emails, since if you lose access to the first email, you still end up getting locked out of your account (at least until a PyPI administrator can intervene). Additionally, this is somewhat surprising behavior, people are going to generally expect that the email is coming to the address they provided.
We should consider sending the password reset to the provided email address instead of the primary address. Of course if someone is reseting by username, we would still send to the primary address in that case.
@pypa/warehouse-committers thoughts?
| I think this makes sense - in fact, that's what I thought was already happening...
https://github.com/pypa/warehouse/blob/master/warehouse/templates/manage/account.html#L192 | 2018-08-17T17:29:07Z | [] | [] |
pypi/warehouse | 4,627 | pypi__warehouse-4627 | [
"4016",
"4016"
] | da5ca10a8f56fd33685ef3c0b46aec89154412f2 | diff --git a/warehouse/legacy/api/json.py b/warehouse/legacy/api/json.py
--- a/warehouse/legacy/api/json.py
+++ b/warehouse/legacy/api/json.py
@@ -41,19 +41,21 @@
"Access-Control-Expose-Headers": ", ".join(["X-PyPI-Last-Serial"]),
}
+_CACHE_DECORATOR = [
+ cache_control(15 * 60), # 15 minutes
+ origin_cache(
+ 1 * 24 * 60 * 60, # 1 day
+ stale_while_revalidate=5 * 60, # 5 minutes
+ stale_if_error=1 * 24 * 60 * 60, # 1 day
+ ),
+]
+
@view_config(
route_name="legacy.api.json.project",
context=Project,
renderer="json",
- decorator=[
- cache_control(15 * 60), # 15 minutes
- origin_cache(
- 1 * 24 * 60 * 60, # 1 day
- stale_while_revalidate=5 * 60, # 5 minutes
- stale_if_error=1 * 24 * 60 * 60, # 1 day
- ),
- ],
+ decorator=_CACHE_DECORATOR,
)
def json_project(project, request):
if project.name != request.matchdict.get("name", project.name):
@@ -75,18 +77,24 @@ def json_project(project, request):
return json_release(release, request)
+@view_config(
+ route_name="legacy.api.json.project_slash",
+ context=Project,
+ decorator=_CACHE_DECORATOR,
+)
+def json_project_slash(project, request):
+ return HTTPMovedPermanently(
+ # Respond with redirect to url without trailing slash
+ request.route_path("legacy.api.json.project", name=project.name),
+ headers=_CORS_HEADERS,
+ )
+
+
@view_config(
route_name="legacy.api.json.release",
context=Release,
renderer="json",
- decorator=[
- cache_control(15 * 60), # 15 minutes
- origin_cache(
- 1 * 24 * 60 * 60, # 1 day
- stale_while_revalidate=5 * 60, # 5 minutes
- stale_if_error=1 * 24 * 60 * 60, # 1 day
- ),
- ],
+ decorator=_CACHE_DECORATOR,
)
def json_release(release, request):
project = release.project
@@ -180,3 +188,18 @@ def json_release(release, request):
"releases": releases,
"last_serial": project.last_serial,
}
+
+
+@view_config(
+ route_name="legacy.api.json.release_slash",
+ context=Release,
+ decorator=_CACHE_DECORATOR,
+)
+def json_release_slash(project, request):
+ return HTTPMovedPermanently(
+ # Respond with redirect to url without trailing slash
+ request.route_path(
+ "legacy.api.json.release", name=project.name, version=project.version
+ ),
+ headers=_CORS_HEADERS,
+ )
diff --git a/warehouse/routes.py b/warehouse/routes.py
--- a/warehouse/routes.py
+++ b/warehouse/routes.py
@@ -221,6 +221,7 @@ def includeme(config):
read_only=True,
domain=warehouse,
)
+
config.add_route(
"legacy.api.json.project",
"/pypi/{name}/json",
@@ -229,6 +230,15 @@ def includeme(config):
read_only=True,
domain=warehouse,
)
+ config.add_route(
+ "legacy.api.json.project_slash",
+ "/pypi/{name}/json/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{name}",
+ read_only=True,
+ domain=warehouse,
+ )
+
config.add_route(
"legacy.api.json.release",
"/pypi/{name}/{version}/json",
@@ -237,6 +247,14 @@ def includeme(config):
read_only=True,
domain=warehouse,
)
+ config.add_route(
+ "legacy.api.json.release_slash",
+ "/pypi/{name}/{version}/json/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{name}/{version}",
+ read_only=True,
+ domain=warehouse,
+ )
# Legacy Action URLs
# TODO: We should probably add Warehouse routes for these that just error
| diff --git a/tests/unit/legacy/api/test_json.py b/tests/unit/legacy/api/test_json.py
--- a/tests/unit/legacy/api/test_json.py
+++ b/tests/unit/legacy/api/test_json.py
@@ -118,6 +118,23 @@ def test_only_prereleases(self, monkeypatch, db_request):
assert json_release.calls == [pretend.call(release, db_request)]
+class TestJSONProjectSlash:
+ def test_normalizing_redirects(self, db_request):
+ project = ProjectFactory.create()
+
+ db_request.route_path = pretend.call_recorder(
+ lambda *a, **kw: "/project/the-redirect"
+ )
+
+ resp = json.json_project_slash(project, db_request)
+
+ assert isinstance(resp, HTTPMovedPermanently)
+ assert db_request.route_path.calls == [
+ pretend.call("legacy.api.json.project", name=project.name)
+ ]
+ assert resp.headers["Location"] == "/project/the-redirect"
+
+
class TestJSONRelease:
def test_normalizing_redirects(self, db_request):
project = ProjectFactory.create()
@@ -428,3 +445,22 @@ def test_minimal_renders(self, pyramid_config, db_request):
],
"last_serial": je.id,
}
+
+
+class TestJSONReleaseSlash:
+ def test_normalizing_redirects(self, db_request):
+ release = ReleaseFactory.create()
+
+ db_request.route_path = pretend.call_recorder(
+ lambda *a, **kw: "/project/the-redirect"
+ )
+
+ resp = json.json_release_slash(release, db_request)
+
+ assert isinstance(resp, HTTPMovedPermanently)
+ assert db_request.route_path.calls == [
+ pretend.call(
+ "legacy.api.json.release", name=release.name, version=release.version
+ )
+ ]
+ assert resp.headers["Location"] == "/project/the-redirect"
diff --git a/tests/unit/test_routes.py b/tests/unit/test_routes.py
--- a/tests/unit/test_routes.py
+++ b/tests/unit/test_routes.py
@@ -243,6 +243,14 @@ def add_policy(name, filename):
read_only=True,
domain=warehouse,
),
+ pretend.call(
+ "legacy.api.json.project_slash",
+ "/pypi/{name}/json/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{name}",
+ read_only=True,
+ domain=warehouse,
+ ),
pretend.call(
"legacy.api.json.release",
"/pypi/{name}/{version}/json",
@@ -251,6 +259,14 @@ def add_policy(name, filename):
read_only=True,
domain=warehouse,
),
+ pretend.call(
+ "legacy.api.json.release_slash",
+ "/pypi/{name}/{version}/json/",
+ factory="warehouse.packaging.models:ProjectFactory",
+ traverse="/{name}/{version}",
+ read_only=True,
+ domain=warehouse,
+ ),
pretend.call("legacy.docs", docs_route_url),
]
| Add trailing slash redirects to JSON API
The JSON API currently resolves URLs like `https://pypi.org/pypi/pip/json`, however adding a trailing slash (e.g. `https://pypi.org/pypi/pip/json/`) results in a 404, even though trailing slashes were valid in legacy PyPI.
PR https://github.com/pypa/warehouse/pull/3971 was an attempt to fix this, but because we have Pyramid configured to automatically add a trailing slash in the event of a 404, this resulted in a redirect loop.
Add trailing slash redirects to JSON API
The JSON API currently resolves URLs like `https://pypi.org/pypi/pip/json`, however adding a trailing slash (e.g. `https://pypi.org/pypi/pip/json/`) results in a 404, even though trailing slashes were valid in legacy PyPI.
PR https://github.com/pypa/warehouse/pull/3971 was an attempt to fix this, but because we have Pyramid configured to automatically add a trailing slash in the event of a 404, this resulted in a redirect loop.
| Is the fix for this to change the Pyramid configuration to special-case this? Or to support `https://pypi.org/pypi/pip/json/` without redirecting?
Is the fix for this to change the Pyramid configuration to special-case this? Or to support `https://pypi.org/pypi/pip/json/` without redirecting? | 2018-08-27T01:50:54Z | [] | [] |
pypi/warehouse | 4,783 | pypi__warehouse-4783 | [
"4680",
"5190"
] | ad6cfe6d8ee78c93aadb76f1361944d3078d049a | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -922,6 +922,12 @@ def file_upload(request):
),
)
+ # Update name if it differs but is still equivalent. We don't need to check if
+ # they are equivalent when normalized because that's already been done when we
+ # queried for the project.
+ if project.name != form.name.data:
+ project.name = form.name.data
+
# Uploading should prevent broken rendered descriptions.
# Temporarily disabled, see
# https://github.com/pypa/warehouse/issues/4079
| diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -2522,6 +2522,59 @@ def test_upload_fails_with_unsupported_wheel_plat(
"400 Binary wheel .* has an unsupported " "platform tag .*", resp.status
)
+ def test_upload_updates_existing_project_name(self, pyramid_config, db_request):
+ pyramid_config.testing_securitypolicy(userid=1)
+
+ user = UserFactory.create()
+ EmailFactory.create(user=user)
+ project = ProjectFactory.create(name="Package-Name")
+ RoleFactory.create(user=user, project=project)
+
+ new_project_name = "package-name"
+ filename = "{}-{}.tar.gz".format(new_project_name, "1.1")
+
+ db_request.user = user
+ db_request.remote_addr = "10.10.10.20"
+ db_request.user_agent = "warehouse-tests/6.6.6"
+ db_request.POST = MultiDict(
+ {
+ "metadata_version": "1.1",
+ "name": new_project_name,
+ "version": "1.1",
+ "summary": "This is my summary!",
+ "filetype": "sdist",
+ "md5_digest": "335c476dc930b959dda9ec82bd65ef19",
+ "content": pretend.stub(
+ filename=filename,
+ file=io.BytesIO(b"A fake file."),
+ type="application/tar",
+ ),
+ }
+ )
+
+ storage_service = pretend.stub(store=lambda path, filepath, meta: None)
+ db_request.find_service = lambda svc, name=None: storage_service
+ db_request.remote_addr = "10.10.10.10"
+ db_request.user_agent = "warehouse-tests/6.6.6"
+
+ resp = legacy.file_upload(db_request)
+
+ assert resp.status_code == 200
+
+ # Ensure that a Project object name has been updated.
+ project = (
+ db_request.db.query(Project).filter(Project.name == new_project_name).one()
+ )
+
+ # Ensure that a Release object has been created.
+ release = (
+ db_request.db.query(Release)
+ .filter((Release.project == project) & (Release.version == "1.1"))
+ .one()
+ )
+
+ assert release.uploaded_via == "warehouse-tests/6.6.6"
+
def test_upload_succeeds_creates_release(self, pyramid_config, db_request):
pyramid_config.testing_securitypolicy(userid=1)
| Update project name on new release if equivalent
Currently a project name is set when the project is created, and cannot be updated.
It should be possible to change the project name to something that will canonicalize to be the same name (e.g. from `CommonMark` to `commonmark`) by uploading a new release with the new name.
---
**Good First Issue**: This issue is good for first time contributors. If you've already contributed to Warehouse, work on [another issue without this label](https://github.com/pypa/warehouse/issues?utf8=%E2%9C%93&q=is%3Aissue+is%3Aopen+-label%3A%22good+first+issue%22) instead. If there is not a corresponding pull request for this issue, it is up for grabs. For directions for getting set up, see our [Getting Started Guide](https://warehouse.pypa.io/development/getting-started/). If you are working on this issue and have questions, feel free to ask them here, [`#pypa-dev` on Freenode](https://webchat.freenode.net/?channels=%23pypa-dev), or the [pypa-dev mailing list](https://groups.google.com/forum/#!forum/pypa-dev).
Possible to rename package due to _ vs - ?
I can't find clear info if renaming of projects like `homeassistant_cli` to `homeassistant-cli` is supported/allowed ?
I tried to update all scripts etc. using _ to - but seems pypi insists on calling it homeassistant_cli because that was what the very first upload by accident had in it.
Can you help rename https://pypi.org/project/homeassistant_cli/ to https://pypi.org/project/homeassistant-cli/ so things are more clean - or is only option to delete the old and create new ?
Thank you!
| Hey @di , can I claim this ticket?
Hi @kwasielew, glad to hear you're working on this. We won't consider an issue "claimed" until there is a PR for it. I don't think anyone else is currently working on this though.
Blocked on #4772.
| 2018-09-28T16:05:32Z | [] | [] |
pypi/warehouse | 4,921 | pypi__warehouse-4921 | [
"4048",
"3607"
] | 797619a89efdb2b009ffc09e5eea0db786ca0652 | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -104,7 +104,7 @@ def namespace_stdlib_list(module_list):
"linux_armv7l",
}
# macosx is a little more complicated:
-_macosx_platform_re = re.compile("macosx_10_(\d+)+_(?P<arch>.*)")
+_macosx_platform_re = re.compile(r"macosx_10_(\d+)+_(?P<arch>.*)")
_macosx_arches = {
"ppc",
"ppc64",
diff --git a/warehouse/migrations/versions/20f4dbe11e9_normalize_function.py b/warehouse/migrations/versions/20f4dbe11e9_normalize_function.py
--- a/warehouse/migrations/versions/20f4dbe11e9_normalize_function.py
+++ b/warehouse/migrations/versions/20f4dbe11e9_normalize_function.py
@@ -25,7 +25,7 @@
def upgrade():
op.execute(
- """
+ r"""
CREATE FUNCTION normalize_pep426_name(text) RETURNS text AS $$
SELECT lower(
regexp_replace(
diff --git a/warehouse/migrations/versions/23a3c4ffe5d_relax_normalization_rules.py b/warehouse/migrations/versions/23a3c4ffe5d_relax_normalization_rules.py
--- a/warehouse/migrations/versions/23a3c4ffe5d_relax_normalization_rules.py
+++ b/warehouse/migrations/versions/23a3c4ffe5d_relax_normalization_rules.py
@@ -28,7 +28,7 @@ def upgrade():
op.execute("DROP INDEX project_name_pep426_normalized")
op.execute(
- """ CREATE OR REPLACE FUNCTION normalize_pep426_name(text)
+ r""" CREATE OR REPLACE FUNCTION normalize_pep426_name(text)
RETURNS text AS
$$
SELECT lower(regexp_replace($1, '(\.|_)', '-', 'ig'))
@@ -42,7 +42,7 @@ def upgrade():
def downgrade():
op.execute(
- """ CREATE OR REPLACE FUNCTION normalize_pep426_name(text)
+ r""" CREATE OR REPLACE FUNCTION normalize_pep426_name(text)
RETURNS text AS
$$
SELECT lower(
diff --git a/warehouse/migrations/versions/3af8d0006ba_normalize_runs_of_characters_to_a_.py b/warehouse/migrations/versions/3af8d0006ba_normalize_runs_of_characters_to_a_.py
--- a/warehouse/migrations/versions/3af8d0006ba_normalize_runs_of_characters_to_a_.py
+++ b/warehouse/migrations/versions/3af8d0006ba_normalize_runs_of_characters_to_a_.py
@@ -26,7 +26,7 @@
def upgrade():
op.execute(
- """ CREATE OR REPLACE FUNCTION normalize_pep426_name(text)
+ r""" CREATE OR REPLACE FUNCTION normalize_pep426_name(text)
RETURNS text AS
$$
SELECT lower(regexp_replace($1, '(\.|_|-)+', '-', 'ig'))
@@ -41,7 +41,7 @@ def upgrade():
def downgrade():
op.execute(
- """ CREATE OR REPLACE FUNCTION normalize_pep426_name(text)
+ r""" CREATE OR REPLACE FUNCTION normalize_pep426_name(text)
RETURNS text AS
$$
SELECT lower(regexp_replace($1, '(\.|_)', '-', 'ig'))
diff --git a/warehouse/migrations/versions/f404a67e0370_disable_legacy_file_types_unless_a_.py b/warehouse/migrations/versions/f404a67e0370_disable_legacy_file_types_unless_a_.py
--- a/warehouse/migrations/versions/f404a67e0370_disable_legacy_file_types_unless_a_.py
+++ b/warehouse/migrations/versions/f404a67e0370_disable_legacy_file_types_unless_a_.py
@@ -26,7 +26,7 @@
def upgrade():
op.execute(
- """ UPDATE packages
+ r""" UPDATE packages
SET allow_legacy_files = 'f'
WHERE name NOT IN (
SELECT DISTINCT ON (packages.name) packages.name
diff --git a/warehouse/routes.py b/warehouse/routes.py
--- a/warehouse/routes.py
+++ b/warehouse/routes.py
@@ -23,7 +23,7 @@ def includeme(config):
# Internal route to make it easier to force a particular status for
# debugging HTTPException templates.
- config.add_route("force-status", "/_force-status/{status:[45]\d\d}/")
+ config.add_route("force-status", r"/_force-status/{status:[45]\d\d}/")
# Basic global routes
config.add_route("index", "/", domain=warehouse)
| diff --git a/tests/unit/accounts/test_forms.py b/tests/unit/accounts/test_forms.py
--- a/tests/unit/accounts/test_forms.py
+++ b/tests/unit/accounts/test_forms.py
@@ -99,7 +99,7 @@ def test_validate_password_disabled_for_compromised_pw(self, db_session):
)
field = pretend.stub(data="pw")
- with pytest.raises(wtforms.validators.ValidationError, match="Bad Password\!"):
+ with pytest.raises(wtforms.validators.ValidationError, match=r"Bad Password\!"):
form.validate_password(field)
assert user_service.find_userid.calls == [pretend.call("my_username")]
diff --git a/tests/unit/legacy/api/test_json.py b/tests/unit/legacy/api/test_json.py
--- a/tests/unit/legacy/api/test_json.py
+++ b/tests/unit/legacy/api/test_json.py
@@ -172,7 +172,7 @@ def test_detail_renders(self, pyramid_config, db_request, db_session):
"telnet,telnet://192.0.2.16:80/",
"urn,urn:oasis:names:specification:docbook:dtd:xml:4.1.2",
"reservedchars,http://example.com?&$+/:;=@#", # Commas don't work!
- "unsafechars,http://example.com <>[]{}|\^%",
+ r"unsafechars,http://example.com <>[]{}|\^%",
]
expected_urls = []
for project_url in reversed(project_urls):
diff --git a/tests/unit/test_routes.py b/tests/unit/test_routes.py
--- a/tests/unit/test_routes.py
+++ b/tests/unit/test_routes.py
@@ -74,7 +74,7 @@ def add_policy(name, filename):
assert config.add_route.calls == [
pretend.call("health", "/_health/"),
- pretend.call("force-status", "/_force-status/{status:[45]\d\d}/"),
+ pretend.call("force-status", r"/_force-status/{status:[45]\d\d}/"),
pretend.call("index", "/", domain=warehouse),
pretend.call("robots.txt", "/robots.txt", domain=warehouse),
pretend.call("opensearch.xml", "/opensearch.xml", domain=warehouse),
diff --git a/tests/unit/utils/test_http.py b/tests/unit/utils/test_http.py
--- a/tests/unit/utils/test_http.py
+++ b/tests/unit/utils/test_http.py
@@ -36,9 +36,9 @@ class TestIsSafeUrl:
r"\/example.com",
r"/\example.com",
"http:///example.com",
- "http:/\//example.com",
- "http:\/example.com",
- "http:/\example.com",
+ r"http:/\//example.com",
+ r"http:\/example.com",
+ r"http:/\example.com",
'javascript:alert("XSS")',
"\njavascript:alert(x)",
"\x08//example.com",
| Update pyflakes to 2.0.0
This PR updates [pyflakes](https://pypi.org/project/pyflakes) from **1.6.0** to **2.0.0**.
<details>
<summary>Changelog</summary>
### 2.0.0
```
- Drop support for EOL Python <2.7 and 3.2-3.3
- Check for unused exception binding in `except:` block
- Handle string literal type annotations
- Ignore redefinitions of `_`, unless originally defined by import
- Support `__class__` without `self` in Python 3
- Issue an error for `raise NotImplemented(...)`
```
</details>
<details>
<summary>Links</summary>
- PyPI: https://pypi.org/project/pyflakes
- Changelog: https://pyup.io/changelogs/pyflakes/
- Repo: https://github.com/PyCQA/pyflakes
</details>
Update pycodestyle to 2.4.0
This PR updates [pycodestyle](https://pypi.org/project/pycodestyle) from **2.3.1** to **2.4.0**.
<details>
<summary>Changelog</summary>
### 2.4.0
```
------------------
New checks:
* Add W504 warning for checking that a break doesn't happen after a binary
operator. This check is ignored by default. PR 502.
* Add W605 warning for invalid escape sequences in string literals. PR 676.
* Add W606 warning for 'async' and 'await' reserved keywords being introduced
in Python 3.7. PR 684.
* Add E252 error for missing whitespace around equal sign in type annotated
function arguments with defaults values. PR 717.
Changes:
* An internal bisect search has replaced a linear search in order to improve
efficiency. PR 648.
* pycodestyle now uses PyPI trove classifiers in order to document supported
python versions on PyPI. PR 654.
* 'setup.cfg' '[wheel]' section has been renamed to '[bdist_wheel]', as
the former is legacy. PR 653.
* pycodestyle now handles very long lines much more efficiently for python
3.2+. Fixes 643. PR 644.
* You can now write 'pycodestyle.StyleGuide(verbose=True)' instead of
'pycodestyle.StyleGuide(verbose=True, paths=['-v'])' in order to achieve
verbosity. PR 663.
* The distribution of pycodestyle now includes the license text in order to
comply with open source licenses which require this. PR 694.
* 'maximum_line_length' now ignores shebang ('!') lines. PR 736.
* Add configuration option for the allowed number of blank lines. It is
implemented as a top level dictionary which can be easily overwritten. Fixes
732. PR 733.
Bugs:
* Prevent a 'DeprecationWarning', and a 'SyntaxError' in future python, caused
by an invalid escape sequence. PR 625.
* Correctly report E501 when the first line of a docstring is too long.
Resolves 622. PR 630.
* Support variable annotation when variable start by a keyword, such as class
variable type annotations in python 3.6. PR 640.
* pycodestyle internals have been changed in order to allow 'python3 -m
cProfile' to report correct metrics. PR 647.
* Fix a spelling mistake in the description of E722. PR 697.
* 'pycodestyle --diff' now does not break if your 'gitconfig' enables
'mnemonicprefix'. PR 706.
```
</details>
<details>
<summary>Links</summary>
- PyPI: https://pypi.org/project/pycodestyle
- Changelog: https://pyup.io/changelogs/pycodestyle/
- Docs: https://pycodestyle.readthedocs.io/
</details>
| Blocked on:
```
flake8 3.5.0 has requirement pyflakes<1.7.0,>=1.5.0, but you have pyflakes 2.0.0.
```
Blocked on:
```
flake8 3.5.0 has requirement pycodestyle<2.4.0,>=2.0.0, but you have pycodestyle 2.4.0.
``` | 2018-10-24T17:13:21Z | [] | [] |
pypi/warehouse | 4,970 | pypi__warehouse-4970 | [
"3127",
"4396"
] | aaea30e44e6fe0b2ac6d948d91f965d03f98d885 | diff --git a/warehouse/manage/views.py b/warehouse/manage/views.py
--- a/warehouse/manage/views.py
+++ b/warehouse/manage/views.py
@@ -39,6 +39,32 @@
from warehouse.utils.project import confirm_project, destroy_docs, remove_project
+def user_projects(request):
+ """ Return all the projects for which the user is a sole owner """
+ projects_owned = (
+ request.db.query(Project)
+ .join(Role.project)
+ .filter(Role.role_name == "Owner", Role.user == request.user)
+ .subquery()
+ )
+
+ with_sole_owner = (
+ request.db.query(Role.package_name)
+ .join(projects_owned)
+ .filter(Role.role_name == "Owner")
+ .group_by(Role.package_name)
+ .having(func.count(Role.package_name) == 1)
+ .subquery()
+ )
+
+ return {
+ "projects_owned": request.db.query(projects_owned).all(),
+ "projects_sole_owned": (
+ request.db.query(Project).join(with_sole_owner).order_by(Project.name).all()
+ ),
+ }
+
+
@view_defaults(
route_name="manage.account",
renderer="manage/account.html",
@@ -57,29 +83,7 @@ def __init__(self, request):
@property
def active_projects(self):
- """ Return all the projects for with the user is a sole owner """
- projects_owned = (
- self.request.db.query(Project)
- .join(Role.project)
- .filter(Role.role_name == "Owner", Role.user == self.request.user)
- .subquery()
- )
-
- with_sole_owner = (
- self.request.db.query(Role.package_name)
- .join(projects_owned)
- .filter(Role.role_name == "Owner")
- .group_by(Role.package_name)
- .having(func.count(Role.package_name) == 1)
- .subquery()
- )
-
- return (
- self.request.db.query(Project)
- .join(with_sole_owner)
- .order_by(Project.name)
- .all()
- )
+ return user_projects(request=self.request)["projects_sole_owned"]
@property
def default_response(self):
@@ -287,19 +291,18 @@ def _key(project):
return project.releases[0].created
return project.created
+ all_user_projects = user_projects(request)
projects_owned = set(
- project.name
- for project in (
- request.db.query(Project.name)
- .join(Role.project)
- .filter(Role.role_name == "Owner", Role.user == request.user)
- .all()
- )
+ project.name for project in all_user_projects["projects_owned"]
+ )
+ projects_sole_owned = set(
+ project.name for project in all_user_projects["projects_sole_owned"]
)
return {
"projects": sorted(request.user.projects, key=_key, reverse=True),
"projects_owned": projects_owned,
+ "projects_sole_owned": projects_sole_owned,
}
| diff --git a/tests/unit/manage/test_views.py b/tests/unit/manage/test_views.py
--- a/tests/unit/manage/test_views.py
+++ b/tests/unit/manage/test_views.py
@@ -100,7 +100,7 @@ def test_active_projects(self, db_request):
# A project with a sole owner that is not the user
not_an_owner = ProjectFactory.create()
- RoleFactory.create(user=user, project=not_an_owner, role_name="Maintatiner")
+ RoleFactory.create(user=user, project=not_an_owner, role_name="Maintainer")
RoleFactory.create(user=another_user, project=not_an_owner, role_name="Owner")
view = views.ManageAccountViews(db_request)
@@ -683,7 +683,12 @@ def test_manage_projects(self, db_request):
older_project_with_no_releases,
]
)
+ user_second_owner = UserFactory(
+ projects=[project_with_older_release, older_project_with_no_releases]
+ )
RoleFactory.create(user=db_request.user, project=project_with_newer_release)
+ RoleFactory.create(user=db_request.user, project=newer_project_with_no_releases)
+ RoleFactory.create(user=user_second_owner, project=project_with_newer_release)
assert views.manage_projects(db_request) == {
"projects": [
@@ -692,7 +697,11 @@ def test_manage_projects(self, db_request):
older_project_with_no_releases,
project_with_older_release,
],
- "projects_owned": {project_with_newer_release.name},
+ "projects_owned": {
+ project_with_newer_release.name,
+ newer_project_with_no_releases.name,
+ },
+ "projects_sole_owned": {newer_project_with_no_releases.name},
}
| Add 'sole owner' badge on list of projects
We've had a lot of positive feedback on the bus factor feature on the account settings page.
It would be nice to also expose this information on `/manage/projects/` so that users don't have to hunt for it :)
---
**Good First Issue**: This issue is good for first time contributors. If you've already contributed to Warehouse, please work on [another issue without this label](https://github.com/pypa/warehouse/issues?utf8=%E2%9C%93&q=is%3Aissue+is%3Aopen+-label%3A%22good+first+issue%22) instead. If there is not a corresponding pull request for this issue, it is up for grabs. For directions for getting set up, see our [Getting Started Guide](https://warehouse.pypa.io/development/getting-started/). If you are working on this issue and have questions, please feel free to ask them here, [`#pypa-dev` on Freenode](https://webchat.freenode.net/?channels=%23pypa-dev), or the [pypa-dev mailing list](https://groups.google.com/forum/#!forum/pypa-dev).
Add sole owner badge on lists of projects
closes #3127
| Something like this using the `<span class="badge>` component would be ideal:

How would you recommend fetching the status for this?
On the account settings page, this is done with a separate query, but I'd be reluctant to add another db query to this frequently used page just for the one badge.
@NotAFile You could reuse the same query, and for each project name, test if it's in the result, and display the badge conditionally.
@di yes, but that was what I was trying to avoid. It's basically two queries on the exact same thing. In django, I'd use the annotate functionality, but this doesn't seem to be available in SQAlchemy. I was wondering how you'd recommend doing this for this project. Two seperate queries and a membership check seems like the worst way to do it, but I might be missing something here.
I would be very surprised if what you want can't be done with SQLAlchemy. Possibly using the SubQuery or Aliasing support.
Can I try this one? @nlhkabu
Yes, sounds great :)
| 2018-10-28T16:59:03Z | [] | [] |
pypi/warehouse | 5,182 | pypi__warehouse-5182 | [
"5124"
] | 390c7dd131ef081d44931e60e25d49c4cfdcb11d | diff --git a/warehouse/accounts/forms.py b/warehouse/accounts/forms.py
--- a/warehouse/accounts/forms.py
+++ b/warehouse/accounts/forms.py
@@ -154,16 +154,24 @@ class NewEmailMixin:
)
def validate_email(self, field):
- if self.user_service.find_userid_by_email(field.data) is not None:
+ userid = self.user_service.find_userid_by_email(field.data)
+
+ if userid and userid == self.user_id:
+ raise wtforms.validators.ValidationError(
+ f"This email address is already being used by this account. "
+ f"Use a different email."
+ )
+ if userid:
raise wtforms.validators.ValidationError(
- "This email address is already being used by another account. "
- "Use a different email."
+ f"This email address is already being used by another account. "
+ f"Use a different email."
)
+
domain = field.data.split("@")[-1]
if domain in disposable_email_domains.blacklist:
raise wtforms.validators.ValidationError(
- "You can't create an account with an email address "
- "from this domain. Use a different email."
+ "You can't use an email address from this domain. Use a "
+ "different email."
)
@@ -193,6 +201,7 @@ class RegistrationForm(
def __init__(self, *args, user_service, **kwargs):
super().__init__(*args, **kwargs)
self.user_service = user_service
+ self.user_id = None
class LoginForm(PasswordMixin, UsernameMixin, forms.Form):
diff --git a/warehouse/manage/forms.py b/warehouse/manage/forms.py
--- a/warehouse/manage/forms.py
+++ b/warehouse/manage/forms.py
@@ -68,9 +68,10 @@ class AddEmailForm(NewEmailMixin, forms.Form):
__params__ = ["email"]
- def __init__(self, *args, user_service, **kwargs):
+ def __init__(self, *args, user_service, user_id, **kwargs):
super().__init__(*args, **kwargs)
self.user_service = user_service
+ self.user_id = user_id
class ChangePasswordForm(PasswordMixin, NewPasswordMixin, forms.Form):
diff --git a/warehouse/manage/views.py b/warehouse/manage/views.py
--- a/warehouse/manage/views.py
+++ b/warehouse/manage/views.py
@@ -106,7 +106,9 @@ def active_projects(self):
def default_response(self):
return {
"save_account_form": SaveAccountForm(name=self.request.user.name),
- "add_email_form": AddEmailForm(user_service=self.user_service),
+ "add_email_form": AddEmailForm(
+ user_service=self.user_service, user_id=self.request.user.id
+ ),
"change_password_form": ChangePasswordForm(
user_service=self.user_service, breach_service=self.breach_service
),
@@ -129,7 +131,11 @@ def save_account(self):
@view_config(request_method="POST", request_param=AddEmailForm.__params__)
def add_email(self):
- form = AddEmailForm(self.request.POST, user_service=self.user_service)
+ form = AddEmailForm(
+ self.request.POST,
+ user_service=self.user_service,
+ user_id=self.request.user.id,
+ )
if form.validate():
email = self.user_service.add_email(self.request.user.id, form.email.data)
| diff --git a/tests/unit/accounts/test_forms.py b/tests/unit/accounts/test_forms.py
--- a/tests/unit/accounts/test_forms.py
+++ b/tests/unit/accounts/test_forms.py
@@ -336,8 +336,8 @@ def test_blacklisted_email_error(self):
assert not form.validate()
assert (
form.email.errors.pop()
- == "You can't create an account with an email address from "
- "this domain. Use a different email."
+ == "You can't use an email address from this domain. Use a "
+ "different email."
)
def test_username_exists(self):
diff --git a/tests/unit/manage/test_forms.py b/tests/unit/manage/test_forms.py
--- a/tests/unit/manage/test_forms.py
+++ b/tests/unit/manage/test_forms.py
@@ -71,10 +71,53 @@ def test_validate_role_name_fails(self, value, expected):
class TestAddEmailForm:
def test_creation(self):
user_service = pretend.stub()
- form = forms.AddEmailForm(user_service=user_service)
+ form = forms.AddEmailForm(user_service=user_service, user_id=pretend.stub())
assert form.user_service is user_service
+ def test_email_exists_error(self):
+ user_id = pretend.stub()
+ form = forms.AddEmailForm(
+ data={"email": "[email protected]"},
+ user_id=user_id,
+ user_service=pretend.stub(find_userid_by_email=lambda _: user_id),
+ )
+
+ assert not form.validate()
+ assert (
+ form.email.errors.pop()
+ == "This email address is already being used by this account. "
+ "Use a different email."
+ )
+
+ def test_email_exists_other_account_error(self):
+ form = forms.AddEmailForm(
+ data={"email": "[email protected]"},
+ user_id=pretend.stub(),
+ user_service=pretend.stub(find_userid_by_email=lambda _: pretend.stub()),
+ )
+
+ assert not form.validate()
+ assert (
+ form.email.errors.pop()
+ == "This email address is already being used by another account. "
+ "Use a different email."
+ )
+
+ def test_blacklisted_email_error(self):
+ form = forms.AddEmailForm(
+ data={"email": "[email protected]"},
+ user_service=pretend.stub(find_userid_by_email=lambda _: None),
+ user_id=pretend.stub(),
+ )
+
+ assert not form.validate()
+ assert (
+ form.email.errors.pop()
+ == "You can't use an email address from this domain. "
+ "Use a different email."
+ )
+
class TestChangePasswordForm:
def test_creation(self):
diff --git a/tests/unit/manage/test_views.py b/tests/unit/manage/test_views.py
--- a/tests/unit/manage/test_views.py
+++ b/tests/unit/manage/test_views.py
@@ -47,12 +47,13 @@ def test_default_response(self, monkeypatch):
breach_service = pretend.stub()
user_service = pretend.stub()
name = pretend.stub()
+ user_id = pretend.stub()
request = pretend.stub(
find_service=lambda iface, **kw: {
IPasswordBreachedService: breach_service,
IUserService: user_service,
}[iface],
- user=pretend.stub(name=name),
+ user=pretend.stub(name=name, id=user_id),
)
save_account_obj = pretend.stub()
save_account_cls = pretend.call_recorder(lambda **kw: save_account_obj)
@@ -79,7 +80,9 @@ def test_default_response(self, monkeypatch):
assert view.request == request
assert view.user_service == user_service
assert save_account_cls.calls == [pretend.call(name=name)]
- assert add_email_cls.calls == [pretend.call(user_service=user_service)]
+ assert add_email_cls.calls == [
+ pretend.call(user_id=user_id, user_service=user_service)
+ ]
assert change_pass_cls.calls == [
pretend.call(user_service=user_service, breach_service=breach_service)
]
@@ -229,7 +232,7 @@ def test_add_email_validation_fails(self, monkeypatch):
db=pretend.stub(flush=lambda: None),
session=pretend.stub(flash=pretend.call_recorder(lambda *a, **kw: None)),
find_service=lambda a, **kw: pretend.stub(),
- user=pretend.stub(emails=[], name=pretend.stub()),
+ user=pretend.stub(emails=[], name=pretend.stub(), id=pretend.stub()),
)
add_email_obj = pretend.stub(
validate=lambda: False, email=pretend.stub(data=email_address)
| Error message is incorrect when attempting to add existing email
If I try to add an email address I've already added to my account, the error message says:
> This email address is already being used by another account. Use a different email.
Which isn't technically true, since the email address is already in use by the _current_ account. We should differentiate it and give a better message when this happens.
---
**Good First Issue**: This issue is good for first time contributors. If you've already contributed to Warehouse, work on [another issue without this label](https://github.com/pypa/warehouse/issues?utf8=%E2%9C%93&q=is%3Aissue+is%3Aopen+-label%3A%22good+first+issue%22) instead. If there is not a corresponding pull request for this issue, it is up for grabs. For directions for getting set up, see our [Getting Started Guide](https://warehouse.pypa.io/development/getting-started/). If you are working on this issue and have questions, feel free to ask them here, [`#pypa-dev` on Freenode](https://webchat.freenode.net/?channels=%23pypa-dev), or the [pypa-dev mailing list](https://groups.google.com/forum/#!forum/pypa-dev).
| 2018-12-11T08:38:07Z | [] | [] |
|
pypi/warehouse | 5,228 | pypi__warehouse-5228 | [
"5085"
] | 1ae184a5aa48bf9a86cdf7c138d5fdc30bbd3804 | diff --git a/warehouse/config.py b/warehouse/config.py
--- a/warehouse/config.py
+++ b/warehouse/config.py
@@ -280,6 +280,7 @@ def configure(settings=None):
filters.setdefault("contains_valid_uris", "warehouse.filters:contains_valid_uris")
filters.setdefault("format_package_type", "warehouse.filters:format_package_type")
filters.setdefault("parse_version", "warehouse.filters:parse_version")
+ filters.setdefault("localize_datetime", "warehouse.filters:localize_datetime")
# We also want to register some global functions for Jinja
jglobals = config.get_settings().setdefault("jinja2.globals", {})
diff --git a/warehouse/filters.py b/warehouse/filters.py
--- a/warehouse/filters.py
+++ b/warehouse/filters.py
@@ -23,6 +23,7 @@
import html5lib.treewalkers
import jinja2
import packaging.version
+import pytz
from pyramid.threadlocal import get_current_request
@@ -154,5 +155,9 @@ def parse_version(version_str):
return packaging.version.parse(version_str)
+def localize_datetime(timestamp):
+ return pytz.utc.localize(timestamp)
+
+
def includeme(config):
config.add_request_method(_camo_url, name="camo_url")
| diff --git a/tests/functional/test_templates.py b/tests/functional/test_templates.py
--- a/tests/functional/test_templates.py
+++ b/tests/functional/test_templates.py
@@ -48,6 +48,7 @@ def test_templates_for_empty_titles():
"contains_valid_uris": "warehouse.filters:contains_valid_uris",
"format_package_type": "warehouse.filters:format_package_type",
"parse_version": "warehouse.filters:parse_version",
+ "localize_datetime": "warehouse.filters:localize_datetime",
}
)
diff --git a/tests/unit/test_filters.py b/tests/unit/test_filters.py
--- a/tests/unit/test_filters.py
+++ b/tests/unit/test_filters.py
@@ -10,6 +10,7 @@
# See the License for the specific language governing permissions and
# limitations under the License.
+import datetime
import urllib.parse
from functools import partial
@@ -186,3 +187,17 @@ def test_format_package_type(inp, expected):
)
def test_parse_version(inp, expected):
assert filters.parse_version(inp) == expected
+
+
[email protected](
+ ("inp", "expected"),
+ [
+ (
+ datetime.datetime(2018, 12, 26, 13, 36, 5, 789013),
+ "2018-12-26 13:36:05.789013 UTC",
+ )
+ ],
+)
+def test_localize_datetime(inp, expected):
+ datetime_format = "%Y-%m-%d %H:%M:%S.%f %Z"
+ assert filters.localize_datetime(inp).strftime(datetime_format) == expected
| Add actual date and time to 'timeago' dates
As per feedback here:
Right now, we display a humanized version of the date / time.
As per feedback here: https://github.com/pypa/warehouse/pull/4402#issuecomment-410383106
> sometimes, I forget when I have released my package since I have 40+ packages. So I resorted to look at pypi for more info. However, github releases and pypi at moment both love moment.js and also give some time ago. Still I could not find the exact date when a version is released.
For this, I think we should add a tooltip that shows *on hover*, e.g.
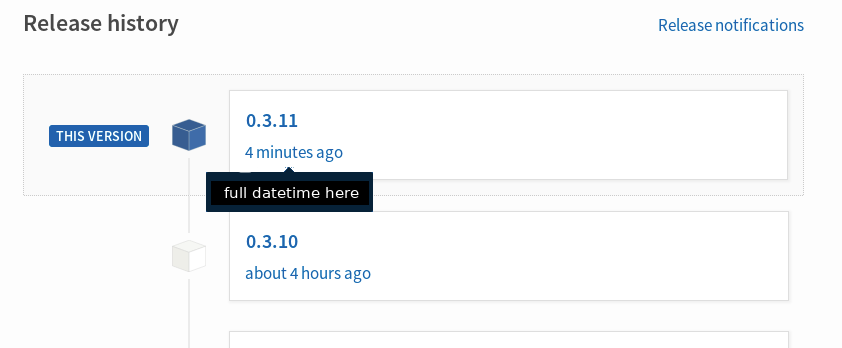
The datetime in the tooltip should follow an international format, e.g `YYYY-MM-DD hh:mm`
this would also solve https://github.com/pypa/warehouse/issues/3010 by providing both a "human friendly" format and technical format.
---
**Good First Issue**: This issue is good for first time contributors. If you've already contributed to Warehouse, work on [another issue without this label](https://github.com/pypa/warehouse/issues?utf8=%E2%9C%93&q=is%3Aissue+is%3Aopen+-label%3A%22good+first+issue%22) instead. If there is not a corresponding pull request for this issue, it is up for grabs. For directions for getting set up, see our [Getting Started Guide](https://warehouse.pypa.io/development/getting-started/). If you are working on this issue and have questions, feel free to ask them here, [`#pypa-dev` on Freenode](https://webchat.freenode.net/?channels=%23pypa-dev), or the [pypa-dev mailing list](https://groups.google.com/forum/#!forum/pypa-dev).
**Screenshot Required**: Please include a screenshot of your update. This helps our team give you feedback faster.
| Hi! This is my first time contributing to warehouse and I'd like to work on this issue.
I couldn't find any project with very recent release history in the dev environment hence there is no "4 min ago" or "about 4 hours ago".
**Screenshot:**
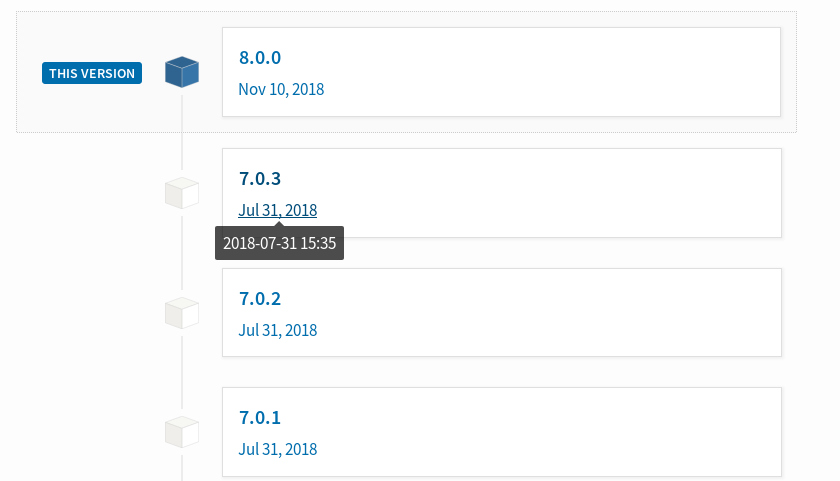
Hey @darkHarry, thanks for working on this. Indeed, the packages in the dev environment will be a few weeks old. You'll need to either:
* manually change the creation date of an existing package via [the interactive shell](https://warehouse.pypa.io/development/getting-started/?highlight=shell#running-the-interactive-shell)
* [upload a new package to your development instance with `twine`](https://warehouse.pypa.io/development/reviewing-patches/?highlight=twine#testing-with-twine)
Let us know if you have any issues with either of these.
(Also, it would be great to include a time zone with the full datetime as well!)
I just checked the Project's release created datetime object and it's tzinfo (time zone info) attribute is None. So, I don't see any way to fetch the time zone. Any other place to look for it?
Pretty sure we store everything in the database using UTC.
Hi @dstufft, so should I add "_UTC_" or "_00:00_" in the date time tooltip? Tell me if I'm missing something : ) | 2018-12-24T08:33:56Z | [] | [] |
pypi/warehouse | 5,626 | pypi__warehouse-5626 | [
"5625"
] | 91cc6f36f03e3f6685374d1983270b9ca74856d3 | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -225,7 +225,10 @@ def _validate_legacy_non_dist_req(requirement):
"Can't direct dependency: {!r}".format(requirement)
)
- if not req.name.isalnum() or req.name[0].isdigit():
+ if any(
+ not identifier.isalnum() or identifier[0].isdigit()
+ for identifier in req.name.split(".")
+ ):
raise wtforms.validators.ValidationError("Use a valid Python identifier.")
| diff --git a/tests/unit/forklift/test_legacy.py b/tests/unit/forklift/test_legacy.py
--- a/tests/unit/forklift/test_legacy.py
+++ b/tests/unit/forklift/test_legacy.py
@@ -97,7 +97,9 @@ def test_validates_invalid_pep440_specifier(self, specifier):
with pytest.raises(ValidationError):
legacy._validate_pep440_specifier(specifier)
- @pytest.mark.parametrize("requirement", ["foo (>=1.0)", "foo", "_foo", "foo2"])
+ @pytest.mark.parametrize(
+ "requirement", ["foo (>=1.0)", "foo", "_foo", "foo2", "foo.bar"]
+ )
def test_validates_legacy_non_dist_req_valid(self, requirement):
legacy._validate_legacy_non_dist_req(requirement)
@@ -111,6 +113,7 @@ def test_validates_legacy_non_dist_req_valid(self, requirement):
"☃ (>=1.0)",
"☃",
"name @ https://github.com/pypa",
+ "foo.2bar",
],
)
def test_validates_legacy_non_dist_req_invalid(self, requirement):
| 400 Client Error: Invalid value for provides
**Describe the bug**
Attempting to upload an updated version of an existing python package results in a HTTPError 400 "Invalid value for provides" response.
A condensed example below.
```python
# Fail
setup(
name='example',
provides=['test.plugins']
)
# Success
setup(
name='example',
provides=['test_plugins']
)
```
**Expected behavior**
The ability to upload a revision to an existing package after bumping the version number in the setup.py file.
**To Reproduce**
```
# Bump version in setup.py file.
$ python setup.py build sdist
$ twine upload -r testpypi dist/*
```
**My Platform**
```
python 2.7.15
twine 1.13.0
```
**Additional context**
<!-- Add any other context, links, etc. about the feature here. -->
The last release of my package was in 2016 and requirements for the provides kwarg could have changed. If that's the case, this can be closed & I'll update my setup.py.
[Pypi release history](https://pypi.org/project/PyUpdater-s3-Plugin/#history) of the package I noticed the issue on.
| 2019-03-26T06:32:45Z | [] | [] |
|
pypi/warehouse | 5,801 | pypi__warehouse-5801 | [
"5769"
] | 7c686fc7c3e2c2752631b497db87a9f570a32ad1 | diff --git a/warehouse/migrations/versions/a9cbb1025607_add_total_size_to_projects.py b/warehouse/migrations/versions/a9cbb1025607_add_total_size_to_projects.py
new file mode 100644
--- /dev/null
+++ b/warehouse/migrations/versions/a9cbb1025607_add_total_size_to_projects.py
@@ -0,0 +1,94 @@
+# Licensed under the Apache License, Version 2.0 (the "License");
+# you may not use this file except in compliance with the License.
+# You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing, software
+# distributed under the License is distributed on an "AS IS" BASIS,
+# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+# See the License for the specific language governing permissions and
+# limitations under the License.
+"""
+add_total_size_to_projects
+
+Revision ID: a9cbb1025607
+Revises: cdb2915fda5c
+Create Date: 2019-06-15 09:01:46.641354
+"""
+
+import sqlalchemy as sa
+
+from alembic import op
+
+revision = "a9cbb1025607"
+down_revision = "cdb2915fda5c"
+
+
+def upgrade():
+ op.add_column(
+ "projects",
+ sa.Column("total_size", sa.BigInteger(), server_default=sa.text("0")),
+ )
+ op.execute(
+ """CREATE OR REPLACE FUNCTION projects_total_size()
+ RETURNS TRIGGER AS $$
+ DECLARE
+ _release_id uuid;
+ _project_id uuid;
+
+ BEGIN
+ IF TG_OP = 'INSERT' THEN
+ _release_id := NEW.release_id;
+ ELSEIF TG_OP = 'UPDATE' THEN
+ _release_id := NEW.release_id;
+ ELSIF TG_OP = 'DELETE' THEN
+ _release_id := OLD.release_id;
+ END IF;
+ _project_id := (SELECT project_id
+ FROM releases
+ WHERE releases.id=_release_id);
+ UPDATE projects
+ SET total_size=t.project_total_size
+ FROM (
+ SELECT SUM(release_files.size) AS project_total_size
+ FROM release_files WHERE release_id IN
+ (SELECT id FROM releases WHERE releases.project_id = _project_id)
+ ) AS t
+ WHERE id=_project_id;
+ RETURN NULL;
+ END;
+ $$ LANGUAGE plpgsql;
+ """
+ )
+ op.execute(
+ """CREATE TRIGGER update_project_total_size
+ AFTER INSERT OR UPDATE OR DELETE ON release_files
+ FOR EACH ROW EXECUTE PROCEDURE projects_total_size();
+ """
+ )
+
+ op.execute(
+ """WITH project_totals AS (
+ SELECT
+ p.id as project_id,
+ sum(size) as project_total
+ FROM
+ release_files rf
+ JOIN releases r on rf.release_id = r.id
+ JOIN projects p on r.project_id = p.id
+ GROUP BY
+ p.id
+ )
+ UPDATE projects AS p
+ SET total_size = project_totals.project_total
+ FROM project_totals
+ WHERE project_totals.project_id = p.id;
+ """
+ )
+
+
+def downgrade():
+ op.execute("DROP TRIGGER update_project_total_size ON release_files;")
+ op.execute("DROP FUNCTION projects_total_size;")
+ op.drop_column("projects", "total_size")
diff --git a/warehouse/packaging/models.py b/warehouse/packaging/models.py
--- a/warehouse/packaging/models.py
+++ b/warehouse/packaging/models.py
@@ -21,6 +21,7 @@
from pyramid.security import Allow
from pyramid.threadlocal import get_current_request
from sqlalchemy import (
+ BigInteger,
Boolean,
CheckConstraint,
Column,
@@ -122,6 +123,8 @@ class Project(SitemapMixin, db.Model):
allow_legacy_files = Column(Boolean, nullable=False, server_default=sql.false())
zscore = Column(Float, nullable=True)
+ total_size = Column(BigInteger, server_default=sql.text("0"))
+
users = orm.relationship(User, secondary=Role.__table__, backref="projects")
releases = orm.relationship(
diff --git a/warehouse/routes.py b/warehouse/routes.py
--- a/warehouse/routes.py
+++ b/warehouse/routes.py
@@ -88,6 +88,12 @@ def includeme(config):
# Search Routes
config.add_route("search", "/search/", domain=warehouse)
+ # Stats Routes
+ config.add_route("stats", "/stats/", accept="text/html", domain=warehouse)
+ config.add_route(
+ "stats.json", "/stats/", accept="application/json", domain=warehouse
+ )
+
# Accounts
config.add_route(
"accounts.profile",
diff --git a/warehouse/views.py b/warehouse/views.py
--- a/warehouse/views.py
+++ b/warehouse/views.py
@@ -40,7 +40,7 @@
from warehouse.accounts import REDIRECT_FIELD_NAME
from warehouse.accounts.models import User
-from warehouse.cache.http import cache_control
+from warehouse.cache.http import add_vary, cache_control
from warehouse.cache.origin import origin_cache
from warehouse.classifiers.models import Classifier
from warehouse.db import DatabaseNotAvailable
@@ -359,6 +359,50 @@ def process_available_filters():
}
+@view_config(
+ route_name="stats",
+ renderer="pages/stats.html",
+ decorator=[
+ add_vary("Accept"),
+ cache_control(1 * 24 * 60 * 60), # 1 day
+ origin_cache(
+ 1 * 24 * 60 * 60, # 1 day
+ stale_while_revalidate=1 * 24 * 60 * 60, # 1 day
+ stale_if_error=1 * 24 * 60 * 60, # 1 day
+ ),
+ ],
+)
+@view_config(
+ route_name="stats.json",
+ renderer="json",
+ decorator=[
+ add_vary("Accept"),
+ cache_control(1 * 24 * 60 * 60), # 1 day
+ origin_cache(
+ 1 * 24 * 60 * 60, # 1 day
+ stale_while_revalidate=1 * 24 * 60 * 60, # 1 day
+ stale_if_error=1 * 24 * 60 * 60, # 1 day
+ ),
+ ],
+ accept="application/json",
+)
+def stats(request):
+ total_size_query = request.db.query(func.sum(Project.total_size)).all()
+ top_100_packages = (
+ request.db.query(Project)
+ .with_entities(Project.name, Project.total_size)
+ .order_by(Project.total_size.desc())
+ .limit(100)
+ .all()
+ )
+ # Move top packages into a dict to make JSON more self describing
+ top_packages = {
+ pkg_name: {"size": pkg_bytes} for pkg_name, pkg_bytes in top_100_packages
+ }
+
+ return {"total_packages_size": total_size_query[0][0], "top_packages": top_packages}
+
+
@view_config(
route_name="includes.current-user-indicator",
renderer="includes/current-user-indicator.html",
| diff --git a/tests/unit/test_routes.py b/tests/unit/test_routes.py
--- a/tests/unit/test_routes.py
+++ b/tests/unit/test_routes.py
@@ -116,6 +116,10 @@ def add_policy(name, filename):
),
pretend.call("classifiers", "/classifiers/", domain=warehouse),
pretend.call("search", "/search/", domain=warehouse),
+ pretend.call("stats", "/stats/", accept="text/html", domain=warehouse),
+ pretend.call(
+ "stats.json", "/stats/", accept="application/json", domain=warehouse
+ ),
pretend.call(
"accounts.profile",
"/user/{username}/",
diff --git a/tests/unit/test_views.py b/tests/unit/test_views.py
--- a/tests/unit/test_views.py
+++ b/tests/unit/test_views.py
@@ -35,6 +35,7 @@
search,
service_unavailable,
session_notifications,
+ stats,
)
from ..common.db.accounts import UserFactory
@@ -583,6 +584,22 @@ def test_classifiers(db_request):
}
+def test_stats(db_request):
+ project = ProjectFactory.create()
+ release1 = ReleaseFactory.create(project=project)
+ release1.created = datetime.date(2011, 1, 1)
+ FileFactory.create(
+ release=release1,
+ filename="{}-{}.tar.gz".format(project.name, release1.version),
+ python_version="source",
+ size=69,
+ )
+ assert stats(db_request) == {
+ "total_packages_size": 69,
+ "top_packages": {project.name: {"size": 69}},
+ }
+
+
def test_health():
request = pretend.stub(
db=pretend.stub(execute=pretend.call_recorder(lambda q: None))
| 503 on /stats route
Previous implementation can be viewed in #5767
The resulting query was causing havoc in the DB, spilling over to disk and taking _a long time_ (minutes).
Suggested approaches:
- Pre calculate results and update on daily basis, serve from some cache
- Add a column on Project model, run a "first pass", and tally as files come in.
| I've never used redis before, but it seems it's just key value store. Does just calculating the data once a day and storing it in some form (e.g. JSON blob) in an async job make sense? Also store the time generated so we can report that so people can see how stale the cached data is?
Then have the /stats endpoint pull the date last generated and stored data and return like we do today. Not running the SQL every time.
cc: @di @dstufft | 2019-05-07T15:40:03Z | [] | [] |
pypi/warehouse | 5,818 | pypi__warehouse-5818 | [
"3739"
] | 212d6385e5d73aaa62444694d02637845d7a51d1 | diff --git a/warehouse/forklift/legacy.py b/warehouse/forklift/legacy.py
--- a/warehouse/forklift/legacy.py
+++ b/warehouse/forklift/legacy.py
@@ -45,6 +45,7 @@
BlacklistedProject,
Dependency,
DependencyKind,
+ Description,
File,
Filename,
JournalEntry,
@@ -52,7 +53,7 @@
Release,
Role,
)
-from warehouse.utils import http
+from warehouse.utils import http, readme
MAX_FILESIZE = 60 * 1024 * 1024 # 60M
MAX_SIGSIZE = 8 * 1024 # 8K
@@ -928,35 +929,38 @@ def file_upload(request):
if project.name != form.name.data:
project.name = form.name.data
- # Uploading should prevent broken rendered descriptions.
- # Temporarily disabled, see
- # https://github.com/pypa/warehouse/issues/4079
- # if form.description.data:
- # description_content_type = form.description_content_type.data
- # if not description_content_type:
- # description_content_type = "text/x-rst"
- # rendered = readme.render(
- # form.description.data, description_content_type, use_fallback=False
- # )
-
- # if rendered is None:
- # if form.description_content_type.data:
- # message = (
- # "The description failed to render "
- # "for '{description_content_type}'."
- # ).format(description_content_type=description_content_type)
- # else:
- # message = (
- # "The description failed to render "
- # "in the default format of reStructuredText."
- # )
- # raise _exc_with_message(
- # HTTPBadRequest,
- # "{message} See {projecthelp} for more information.".format(
- # message=message,
- # projecthelp=request.help_url(_anchor="description-content-type"),
- # ),
- # ) from None
+ # Render our description so we can save from having to render this data every time
+ # we load a project description page.
+ rendered = None
+ if form.description.data:
+ description_content_type = form.description_content_type.data
+ # if not description_content_type:
+ # description_content_type = "text/x-rst"
+ rendered = readme.render(
+ form.description.data, description_content_type # use_fallback=False
+ )
+
+ # Uploading should prevent broken rendered descriptions.
+ # Temporarily disabled, see
+ # https://github.com/pypa/warehouse/issues/4079
+ # if rendered is None:
+ # if form.description_content_type.data:
+ # message = (
+ # "The description failed to render "
+ # "for '{description_content_type}'."
+ # ).format(description_content_type=description_content_type)
+ # else:
+ # message = (
+ # "The description failed to render "
+ # "in the default format of reStructuredText."
+ # )
+ # raise _exc_with_message(
+ # HTTPBadRequest,
+ # "{message} See {projecthelp} for more information.".format(
+ # message=message,
+ # projecthelp=request.help_url(_anchor="description-content-type"),
+ # ),
+ # ) from None
try:
canonical_version = packaging.utils.canonicalize_version(form.version.data)
@@ -1001,6 +1005,12 @@ def file_upload(request):
)
),
canonical_version=canonical_version,
+ description=Description(
+ content_type=form.description_content_type.data,
+ raw=form.description.data or "",
+ html=rendered or "",
+ rendered_by=readme.renderer_version(),
+ ),
**{
k: getattr(form, k).data
for k in {
@@ -1008,8 +1018,6 @@ def file_upload(request):
# should pull off and insert into our new release.
"version",
"summary",
- "description",
- "description_content_type",
"license",
"author",
"author_email",
diff --git a/warehouse/legacy/api/json.py b/warehouse/legacy/api/json.py
--- a/warehouse/legacy/api/json.py
+++ b/warehouse/legacy/api/json.py
@@ -157,8 +157,8 @@ def json_release(release, request):
"name": project.name,
"version": release.version,
"summary": release.summary,
- "description_content_type": release.description_content_type,
- "description": release.description,
+ "description_content_type": release.description.content_type,
+ "description": release.description.raw,
"keywords": release.keywords,
"license": release.license,
"classifiers": list(release.classifiers),
diff --git a/warehouse/legacy/api/xmlrpc/views.py b/warehouse/legacy/api/xmlrpc/views.py
--- a/warehouse/legacy/api/xmlrpc/views.py
+++ b/warehouse/legacy/api/xmlrpc/views.py
@@ -359,7 +359,7 @@ def release_data(request, package_name: str, version: str):
try:
release = (
request.db.query(Release)
- .options(orm.undefer("description"))
+ .options(orm.joinedload(Release.description))
.join(Project)
.filter(
(Project.normalized_name == func.normalize_pep426_name(package_name))
@@ -390,7 +390,7 @@ def release_data(request, package_name: str, version: str):
"maintainer": _clean_for_xml(release.maintainer),
"maintainer_email": _clean_for_xml(release.maintainer_email),
"summary": _clean_for_xml(release.summary),
- "description": _clean_for_xml(release.description),
+ "description": _clean_for_xml(release.description.raw),
"license": _clean_for_xml(release.license),
"keywords": _clean_for_xml(release.keywords),
"platform": release.platform,
diff --git a/warehouse/migrations/versions/9ca7d5668af4_refactor_description_to_its_own_table.py b/warehouse/migrations/versions/9ca7d5668af4_refactor_description_to_its_own_table.py
new file mode 100644
--- /dev/null
+++ b/warehouse/migrations/versions/9ca7d5668af4_refactor_description_to_its_own_table.py
@@ -0,0 +1,84 @@
+# Licensed under the Apache License, Version 2.0 (the "License");
+# you may not use this file except in compliance with the License.
+# You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing, software
+# distributed under the License is distributed on an "AS IS" BASIS,
+# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+# See the License for the specific language governing permissions and
+# limitations under the License.
+"""
+Refactor description to its own table
+
+Revision ID: 9ca7d5668af4
+Revises: 42f0409bb702
+Create Date: 2019-05-10 16:19:04.008388
+"""
+
+import sqlalchemy as sa
+
+from alembic import op
+from sqlalchemy.dialects import postgresql
+
+revision = "9ca7d5668af4"
+down_revision = "42f0409bb702"
+
+
+def upgrade():
+ op.create_table(
+ "release_descriptions",
+ sa.Column(
+ "id",
+ postgresql.UUID(as_uuid=True),
+ server_default=sa.text("gen_random_uuid()"),
+ nullable=False,
+ ),
+ sa.Column("content_type", sa.Text(), nullable=True),
+ sa.Column("raw", sa.Text(), nullable=False),
+ sa.Column("html", sa.Text(), nullable=False),
+ sa.Column("rendered_by", sa.Text(), nullable=False),
+ sa.Column("release_id", postgresql.UUID(as_uuid=True), nullable=False),
+ sa.PrimaryKeyConstraint("id"),
+ )
+ op.add_column(
+ "releases",
+ sa.Column("description_id", postgresql.UUID(as_uuid=True), nullable=True),
+ )
+ op.create_foreign_key(
+ None,
+ "releases",
+ "release_descriptions",
+ ["description_id"],
+ ["id"],
+ onupdate="CASCADE",
+ ondelete="CASCADE",
+ )
+
+ # Backfill our data into the description table.
+ op.execute(
+ """ WITH inserted_descriptions AS (
+ INSERT INTO release_descriptions
+ (content_type, raw, html, rendered_by, release_id)
+ SELECT
+ description_content_type, COALESCE(description, ''), '', '', id
+ FROM releases
+ RETURNING release_id, id AS description_id
+ )
+ UPDATE releases
+ SET description_id = ids.description_id
+ FROM inserted_descriptions AS ids
+ WHERE id = release_id
+ """
+ )
+
+ op.alter_column("releases", "description_id", nullable=False)
+
+ op.drop_column("releases", "description_content_type")
+ op.drop_column("releases", "description")
+ op.drop_column("release_descriptions", "release_id")
+
+
+def downgrade():
+ raise RuntimeError(f"Cannot downgrade past revision: {revision!r}")
diff --git a/warehouse/packaging/__init__.py b/warehouse/packaging/__init__.py
--- a/warehouse/packaging/__init__.py
+++ b/warehouse/packaging/__init__.py
@@ -18,7 +18,7 @@
from warehouse.cache.origin import key_factory, receive_set
from warehouse.packaging.interfaces import IDocsStorage, IFileStorage
from warehouse.packaging.models import File, Project, Release, Role
-from warehouse.packaging.tasks import compute_trending
+from warehouse.packaging.tasks import compute_trending, update_description_html
@db.listens_for(User.name, "set")
@@ -89,6 +89,8 @@ def includeme(config):
],
)
+ config.add_periodic_task(crontab(minute="*/5"), update_description_html)
+
# Add a periodic task to compute trending once a day, assuming we have
# been configured to be able to access BigQuery.
if config.get_settings().get("warehouse.trending_table"):
diff --git a/warehouse/packaging/models.py b/warehouse/packaging/models.py
--- a/warehouse/packaging/models.py
+++ b/warehouse/packaging/models.py
@@ -257,6 +257,16 @@ def _dependency_relation(kind):
)
+class Description(db.Model):
+
+ __tablename__ = "release_descriptions"
+
+ content_type = Column(Text)
+ raw = Column(Text, nullable=False)
+ html = Column(Text, nullable=False)
+ rendered_by = Column(Text, nullable=False)
+
+
class Release(db.Model):
__tablename__ = "releases"
@@ -287,7 +297,6 @@ def __table_args__(cls): # noqa
home_page = Column(Text)
license = Column(Text)
summary = Column(Text)
- description_content_type = Column(Text)
keywords = Column(Text)
platform = Column(Text)
download_url = Column(Text)
@@ -297,14 +306,21 @@ def __table_args__(cls): # noqa
DateTime(timezone=False), nullable=False, server_default=sql.func.now()
)
- # We defer this column because it is a very large column (it can be MB in
- # size) and we very rarely actually want to access it. Typically we only
- # need it when rendering the page for a single project, but many of our
- # queries only need to access a few of the attributes of a Release. Instead
- # of playing whack-a-mole and using load_only() or defer() on each of
- # those queries, deferring this here makes the default case more
- # performant.
- description = orm.deferred(Column(Text))
+ description_id = Column(
+ ForeignKey("release_descriptions.id", onupdate="CASCADE", ondelete="CASCADE"),
+ nullable=False,
+ )
+ description = orm.relationship(
+ "Description",
+ backref=orm.backref(
+ "release",
+ cascade="all, delete-orphan",
+ passive_deletes=True,
+ passive_updates=True,
+ single_parent=True,
+ uselist=False,
+ ),
+ )
_classifiers = orm.relationship(
Classifier,
diff --git a/warehouse/packaging/tasks.py b/warehouse/packaging/tasks.py
--- a/warehouse/packaging/tasks.py
+++ b/warehouse/packaging/tasks.py
@@ -12,7 +12,8 @@
from warehouse import tasks
from warehouse.cache.origin import IOriginCache
-from warehouse.packaging.models import Project
+from warehouse.packaging.models import Description, Project
+from warehouse.utils import readme
@tasks.task(ignore_result=True, acks_late=True)
@@ -94,3 +95,19 @@ def compute_trending(request):
pass
else:
cacher.purge(["trending"])
+
+
[email protected](ignore_result=True, acks_late=True)
+def update_description_html(request):
+ renderer_version = readme.renderer_version()
+
+ descriptions = (
+ request.db.query(Description)
+ .filter(Description.rendered_by != renderer_version)
+ .yield_per(100)
+ .limit(5000)
+ )
+
+ for description in descriptions:
+ description.html = readme.render(description.raw, description.content_type)
+ description.rendered_by = renderer_version
diff --git a/warehouse/packaging/views.py b/warehouse/packaging/views.py
--- a/warehouse/packaging/views.py
+++ b/warehouse/packaging/views.py
@@ -77,8 +77,16 @@ def release_detail(release, request):
if project.name != request.matchdict.get("name", project.name):
return HTTPMovedPermanently(request.current_route_path(name=project.name))
- # Render the release description.
- description = readme.render(release.description, release.description_content_type)
+ # Grab the rendered description if it exists, and if it doesn't, then we will render
+ # it inline.
+ # TODO: Remove the fallback to rendering inline and only support displaying the
+ # already rendered content.
+ if release.description.html:
+ description = release.description.html
+ else:
+ description = readme.render(
+ release.description.raw, release.description.content_type
+ )
# Get all of the maintainers for this project.
maintainers = [
diff --git a/warehouse/search/tasks.py b/warehouse/search/tasks.py
--- a/warehouse/search/tasks.py
+++ b/warehouse/search/tasks.py
@@ -24,7 +24,13 @@
from sqlalchemy.orm import aliased
from warehouse import tasks
-from warehouse.packaging.models import Classifier, Project, Release, release_classifiers
+from warehouse.packaging.models import (
+ Classifier,
+ Description,
+ Project,
+ Release,
+ release_classifiers,
+)
from warehouse.packaging.search import Project as ProjectDocument
from warehouse.search.utils import get_index
from warehouse.utils.db import windowed_query
@@ -69,7 +75,7 @@ def _project_docs(db, project_name=None):
release_data = (
db.query(
- Release.description,
+ Description.raw.label("description"),
Release.version.label("latest_version"),
all_versions,
Release.author,
@@ -89,6 +95,7 @@ def _project_docs(db, project_name=None):
)
.select_from(releases_list)
.join(Release, Release.id == releases_list.c.id)
+ .join(Description)
.outerjoin(Release.project)
)
| diff --git a/tests/common/db/packaging.py b/tests/common/db/packaging.py
--- a/tests/common/db/packaging.py
+++ b/tests/common/db/packaging.py
@@ -22,12 +22,14 @@
BlacklistedProject,
Dependency,
DependencyKind,
+ Description,
File,
JournalEntry,
Project,
Release,
Role,
)
+from warehouse.utils import readme
from .accounts import UserFactory
from .base import WarehouseFactory
@@ -41,6 +43,16 @@ class Meta:
name = factory.fuzzy.FuzzyText(length=12)
+class DescriptionFactory(WarehouseFactory):
+ class Meta:
+ model = Description
+
+ id = factory.LazyFunction(uuid.uuid4)
+ raw = factory.fuzzy.FuzzyText(length=100)
+ html = factory.LazyAttribute(lambda o: readme.render(o.raw))
+ rendered_by = factory.LazyAttribute(lambda o: readme.renderer_version())
+
+
class ReleaseFactory(WarehouseFactory):
class Meta:
model = Release
@@ -54,6 +66,7 @@ class Meta:
_pypi_ordering = factory.Sequence(lambda n: n)
uploader = factory.SubFactory(UserFactory)
+ description = factory.SubFactory(DescriptionFactory)
class FileFactory(WarehouseFactory):
diff --git a/tests/unit/legacy/api/test_json.py b/tests/unit/legacy/api/test_json.py
--- a/tests/unit/legacy/api/test_json.py
+++ b/tests/unit/legacy/api/test_json.py
@@ -21,6 +21,7 @@
from ....common.db.accounts import UserFactory
from ....common.db.packaging import (
+ DescriptionFactory,
FileFactory,
JournalEntryFactory,
ProjectFactory,
@@ -187,7 +188,9 @@ def test_detail_renders(self, pyramid_config, db_request, db_session):
ReleaseFactory.create(
project=project,
version="3.0",
- description_content_type=description_content_type,
+ description=DescriptionFactory.create(
+ content_type=description_content_type
+ ),
)
]
@@ -239,7 +242,7 @@ def test_detail_renders(self, pyramid_config, db_request, db_session):
"bugtrack_url": None,
"classifiers": [],
"description_content_type": description_content_type,
- "description": None,
+ "description": releases[-1].description.raw,
"docs_url": "/the/fake/url/",
"download_url": None,
"downloads": {"last_day": -1, "last_week": -1, "last_month": -1},
@@ -384,8 +387,8 @@ def test_minimal_renders(self, pyramid_config, db_request):
"author_email": None,
"bugtrack_url": None,
"classifiers": [],
- "description_content_type": None,
- "description": None,
+ "description_content_type": release.description.content_type,
+ "description": release.description.raw,
"docs_url": None,
"download_url": None,
"downloads": {"last_day": -1, "last_week": -1, "last_month": -1},
diff --git a/tests/unit/legacy/api/xmlrpc/test_xmlrpc.py b/tests/unit/legacy/api/xmlrpc/test_xmlrpc.py
--- a/tests/unit/legacy/api/xmlrpc/test_xmlrpc.py
+++ b/tests/unit/legacy/api/xmlrpc/test_xmlrpc.py
@@ -605,7 +605,7 @@ def test_release_data(db_request):
"maintainer": release.maintainer,
"maintainer_email": release.maintainer_email,
"summary": release.summary,
- "description": release.description,
+ "description": release.description.raw,
"license": release.license,
"keywords": release.keywords,
"platform": release.platform,
diff --git a/tests/unit/packaging/test_init.py b/tests/unit/packaging/test_init.py
--- a/tests/unit/packaging/test_init.py
+++ b/tests/unit/packaging/test_init.py
@@ -19,7 +19,7 @@
from warehouse.accounts.models import Email, User
from warehouse.packaging.interfaces import IDocsStorage, IFileStorage
from warehouse.packaging.models import File, Project, Release, Role
-from warehouse.packaging.tasks import compute_trending
+from warehouse.packaging.tasks import compute_trending, update_description_html
@pytest.mark.parametrize("with_trending", [True, False])
@@ -106,5 +106,10 @@ def key_factory(keystring, iterate_on=None):
if with_trending:
assert config.add_periodic_task.calls == [
- pretend.call(crontab(minute=0, hour=3), compute_trending)
+ pretend.call(crontab(minute="*/5"), update_description_html),
+ pretend.call(crontab(minute=0, hour=3), compute_trending),
+ ]
+ else:
+ assert config.add_periodic_task.calls == [
+ pretend.call(crontab(minute="*/5"), update_description_html)
]
diff --git a/tests/unit/packaging/test_tasks.py b/tests/unit/packaging/test_tasks.py
--- a/tests/unit/packaging/test_tasks.py
+++ b/tests/unit/packaging/test_tasks.py
@@ -16,10 +16,11 @@
from google.cloud.bigquery import Row
from warehouse.cache.origin import IOriginCache
-from warehouse.packaging.models import Project
-from warehouse.packaging.tasks import compute_trending
+from warehouse.packaging.models import Description, Project
+from warehouse.packaging.tasks import compute_trending, update_description_html
+from warehouse.utils import readme
-from ...common.db.packaging import ProjectFactory
+from ...common.db.packaging import DescriptionFactory, ProjectFactory
class TestComputeTrending:
@@ -108,3 +109,28 @@ def find_service(iface=None, name=None):
projects[1].name: 2,
projects[2].name: -1,
}
+
+
+def test_update_description_html(monkeypatch, db_request):
+ current_version = "24.0"
+ previous_version = "23.0"
+
+ monkeypatch.setattr(readme, "renderer_version", lambda: current_version)
+
+ descriptions = [
+ DescriptionFactory.create(html="rendered", rendered_by=current_version),
+ DescriptionFactory.create(html="not this one", rendered_by=previous_version),
+ DescriptionFactory.create(html="", rendered_by=""), # Initial migration state
+ ]
+
+ update_description_html(db_request)
+
+ assert set(
+ db_request.db.query(
+ Description.raw, Description.html, Description.rendered_by
+ ).all()
+ ) == {
+ (descriptions[0].raw, "rendered", current_version),
+ (descriptions[1].raw, readme.render(descriptions[1].raw), current_version),
+ (descriptions[2].raw, readme.render(descriptions[2].raw), current_version),
+ }
diff --git a/tests/unit/packaging/test_views.py b/tests/unit/packaging/test_views.py
--- a/tests/unit/packaging/test_views.py
+++ b/tests/unit/packaging/test_views.py
@@ -21,6 +21,7 @@
from ...common.db.accounts import UserFactory
from ...common.db.classifiers import ClassifierFactory
from ...common.db.packaging import (
+ DescriptionFactory,
FileFactory,
ProjectFactory,
ReleaseFactory,
@@ -145,6 +146,52 @@ def test_normalizing_version_redirects(self, db_request):
pretend.call(name=release.project.name, version=release.version)
]
+ def test_detail_rendered(self, db_request):
+ users = [UserFactory.create(), UserFactory.create(), UserFactory.create()]
+ project = ProjectFactory.create()
+ releases = [
+ ReleaseFactory.create(
+ project=project,
+ version=v,
+ description=DescriptionFactory.create(
+ raw="unrendered description",
+ html="rendered description",
+ content_type="text/plain",
+ ),
+ )
+ for v in ["1.0", "2.0", "3.0", "4.0.dev0"]
+ ]
+ files = [
+ FileFactory.create(
+ release=r,
+ filename="{}-{}.tar.gz".format(project.name, r.version),
+ python_version="source",
+ )
+ for r in releases
+ ]
+
+ # Create a role for each user
+ for user in users:
+ RoleFactory.create(user=user, project=project)
+
+ # Add an extra role for one user, to ensure deduplication
+ RoleFactory.create(user=users[0], project=project, role_name="another role")
+
+ result = views.release_detail(releases[1], db_request)
+
+ assert result == {
+ "project": project,
+ "release": releases[1],
+ "files": [files[1]],
+ "description": "rendered description",
+ "latest_version": project.latest_version,
+ "all_versions": [
+ (r.version, r.created, r.is_prerelease) for r in reversed(releases)
+ ],
+ "maintainers": sorted(users, key=lambda u: u.username.lower()),
+ "license": None,
+ }
+
def test_detail_renders(self, monkeypatch, db_request):
users = [UserFactory.create(), UserFactory.create(), UserFactory.create()]
project = ProjectFactory.create()
@@ -152,8 +199,9 @@ def test_detail_renders(self, monkeypatch, db_request):
ReleaseFactory.create(
project=project,
version=v,
- description="unrendered description",
- description_content_type="text/plain",
+ description=DescriptionFactory.create(
+ raw="unrendered description", html="", content_type="text/plain"
+ ),
)
for v in ["1.0", "2.0", "3.0", "4.0.dev0"]
]
diff --git a/tests/unit/search/test_tasks.py b/tests/unit/search/test_tasks.py
--- a/tests/unit/search/test_tasks.py
+++ b/tests/unit/search/test_tasks.py
@@ -55,6 +55,9 @@ def test_project_docs(db_session):
"normalized_name": p.normalized_name,
"version": [r.version for r in prs],
"latest_version": first(prs, key=lambda r: not r.is_prerelease).version,
+ "description": first(
+ prs, key=lambda r: not r.is_prerelease
+ ).description.raw,
},
}
for p, prs in sorted(releases.items(), key=lambda x: x[0].id)
@@ -82,6 +85,9 @@ def test_single_project_doc(db_session):
"normalized_name": p.normalized_name,
"version": [r.version for r in prs],
"latest_version": first(prs, key=lambda r: not r.is_prerelease).version,
+ "description": first(
+ prs, key=lambda r: not r.is_prerelease
+ ).description.raw,
},
}
for p, prs in sorted(releases.items(), key=lambda x: x[0].name.lower())
| cache/stash long_descriptions rendered by readme_render
RE https://github.com/pypa/readme_renderer/issues/95
A handful of long descriptions have _long_ render times, such as https://gitlab.com/hansroh/skitai/blob/master/README.rst.
A proposed solution:
- Store `long_description` as usual at upload time.
- Call a task to attempt to render the long description.
- Task renders long_description and stores resulting html with the version number of readme_renderer
- When rendering a project page, check for rendered version and fallback to attempting render
- On readme_renderer upgrade, have some mechanism to rerender all readmes via the same celery task as above.
| cc @theacodes
Research notes (for my own benefit/for the benefit of whoever takes this on):
1. Current un-rendered `description` field is [here](https://github.com/pypa/warehouse/blob/7947068a27fd9184d93a2089b882656fb60d2704/warehouse/packaging/models.py#L338).
2. The description is only rendered for HTML views and is done in the template [here](https://github.com/pypa/warehouse/blob/b86fe0351cdbaacb36a5d8c080a1a380300aa4c5/warehouse/templates/packaging/detail.html#L266) using the `readme` filter.
3. The filter `readme` is defined [here](https://github.com/pypa/warehouse/blob/b86fe0351cdbaacb36a5d8c080a1a380300aa4c5/warehouse/filters.py#L75).
For the benefit of accomplishing this task and testability, we should likely pull the rendering logic out of the context filter and into its own thing (not sure where they would live yet) and just have the filter be a thin wrapper. We could then use the common function from both the task and the templates.
Might not even be necessary to keep it as a filter, it could be passed into the template from the view instead, which would make it a little easier to determine a cache key.
That could definitely work. | 2019-05-08T15:06:32Z | [] | [] |
pypi/warehouse | 5,889 | pypi__warehouse-5889 | [
"5866"
] | 3171d72c5d103f5bfa0e46c6c5c2bb4149fa274a | diff --git a/warehouse/accounts/interfaces.py b/warehouse/accounts/interfaces.py
--- a/warehouse/accounts/interfaces.py
+++ b/warehouse/accounts/interfaces.py
@@ -103,14 +103,16 @@ def is_disabled(user_id):
def has_two_factor(user_id):
"""
- Returns True if the user has two factor authentication.
+ Returns True if the user has any form of two factor
+ authentication and is allowed to use it.
"""
def get_totp_secret(user_id):
"""
Returns the user's TOTP secret as bytes.
- If the user doesn't have a TOTP secret, returns None.
+ If the user doesn't have a TOTP secret or is not
+ allowed to use a second factor, returns None.
"""
def check_totp_value(user_id, totp_value, *, tags=None):
diff --git a/warehouse/accounts/models.py b/warehouse/accounts/models.py
--- a/warehouse/accounts/models.py
+++ b/warehouse/accounts/models.py
@@ -80,7 +80,6 @@ class User(SitemapMixin, db.Model):
Enum(DisableReason, values_callable=lambda x: [e.value for e in x]),
nullable=True,
)
- two_factor_allowed = Column(Boolean, nullable=False, server_default=sql.false())
totp_secret = Column(Binary(length=20), nullable=True)
emails = orm.relationship(
@@ -110,7 +109,11 @@ def email(self):
def has_two_factor(self):
# TODO: This is where user.u2f_provisioned et al.
# will also go.
- return self.two_factor_allowed and self.totp_secret is not None
+ return self.totp_secret is not None
+
+ @property
+ def two_factor_provisioning_allowed(self):
+ return self.primary_email is not None and self.primary_email.verified
class UnverifyReasons(enum.Enum):
diff --git a/warehouse/accounts/services.py b/warehouse/accounts/services.py
--- a/warehouse/accounts/services.py
+++ b/warehouse/accounts/services.py
@@ -231,7 +231,7 @@ def is_disabled(self, user_id):
def has_two_factor(self, user_id):
"""
Returns True if the user has any form of two factor
- authentication.
+ authentication and is allowed to use it.
"""
user = self.get_user(user_id)
@@ -241,21 +241,18 @@ def get_totp_secret(self, user_id):
"""
Returns the user's TOTP secret as bytes.
- If the user doesn't have a TOTP secret, returns None.
+ If the user doesn't have a TOTP, returns None.
"""
user = self.get_user(user_id)
- # TODO: Remove once all users are allowed to enroll in two-factor.
- if not user.two_factor_allowed: # pragma: no cover
- return None
-
return user.totp_secret
def check_totp_value(self, user_id, totp_value, *, tags=None):
"""
Returns True if the given TOTP is valid against the user's secret.
- If the user doesn't have a TOTP secret, returns False.
+ If the user doesn't have a TOTP secret or isn't allowed
+ to use second factor methods, returns False.
"""
tags = tags if tags is not None else []
self._metrics.increment("warehouse.authentication.two_factor.start", tags=tags)
diff --git a/warehouse/admin/views/users.py b/warehouse/admin/views/users.py
--- a/warehouse/admin/views/users.py
+++ b/warehouse/admin/views/users.py
@@ -84,8 +84,6 @@ class UserForm(forms.Form):
validators=[wtforms.validators.Optional(), wtforms.validators.Length(max=100)]
)
- two_factor_allowed = wtforms.fields.BooleanField()
-
is_active = wtforms.fields.BooleanField()
is_superuser = wtforms.fields.BooleanField()
is_moderator = wtforms.fields.BooleanField()
diff --git a/warehouse/manage/views.py b/warehouse/manage/views.py
--- a/warehouse/manage/views.py
+++ b/warehouse/manage/views.py
@@ -325,6 +325,12 @@ def default_response(self):
@view_config(route_name="manage.account.totp-provision.image", request_method="GET")
def generate_totp_qr(self):
+ if not self.request.user.two_factor_provisioning_allowed:
+ self.request.session.flash(
+ "Modifying 2FA requires a verified email.", queue="error"
+ )
+ return Response(status=403)
+
totp_secret = self.user_service.get_totp_secret(self.request.user.id)
if totp_secret:
return Response(status=403)
@@ -337,6 +343,12 @@ def generate_totp_qr(self):
@view_config(request_method="GET")
def totp_provision(self):
+ if not self.request.user.two_factor_provisioning_allowed:
+ self.request.session.flash(
+ "Modifying 2FA requires a verified email.", queue="error"
+ )
+ return Response(status=403)
+
totp_secret = self.user_service.get_totp_secret(self.request.user.id)
if totp_secret:
self.request.session.flash("TOTP already provisioned.", queue="error")
@@ -346,6 +358,12 @@ def totp_provision(self):
@view_config(request_method="POST", request_param=ProvisionTOTPForm.__params__)
def validate_totp_provision(self):
+ if not self.request.user.two_factor_provisioning_allowed:
+ self.request.session.flash(
+ "Modifying 2FA requires a verified email.", queue="error"
+ )
+ return Response(status=403)
+
totp_secret = self.user_service.get_totp_secret(self.request.user.id)
if totp_secret:
self.request.session.flash("TOTP already provisioned.", queue="error")
@@ -371,6 +389,12 @@ def validate_totp_provision(self):
@view_config(request_method="POST", request_param=DeleteTOTPForm.__params__)
def delete_totp(self):
+ if not self.request.user.two_factor_provisioning_allowed:
+ self.request.session.flash(
+ "Modifying 2FA requires a verified email.", queue="error"
+ )
+ return Response(status=403)
+
totp_secret = self.user_service.get_totp_secret(self.request.user.id)
if not totp_secret:
self.request.session.flash("No TOTP application to delete.", queue="error")
diff --git a/warehouse/migrations/versions/e1b493d3b171_remove_two_factor_allowed.py b/warehouse/migrations/versions/e1b493d3b171_remove_two_factor_allowed.py
new file mode 100644
--- /dev/null
+++ b/warehouse/migrations/versions/e1b493d3b171_remove_two_factor_allowed.py
@@ -0,0 +1,42 @@
+# Licensed under the Apache License, Version 2.0 (the "License");
+# you may not use this file except in compliance with the License.
+# You may obtain a copy of the License at
+#
+# http://www.apache.org/licenses/LICENSE-2.0
+#
+# Unless required by applicable law or agreed to in writing, software
+# distributed under the License is distributed on an "AS IS" BASIS,
+# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
+# See the License for the specific language governing permissions and
+# limitations under the License.
+"""
+remove two_factor_allowed
+
+Revision ID: e1b493d3b171
+Revises: 9ca7d5668af4
+Create Date: 2019-05-20 20:39:28.616037
+"""
+
+import sqlalchemy as sa
+
+from alembic import op
+
+revision = "e1b493d3b171"
+down_revision = "9ca7d5668af4"
+
+
+def upgrade():
+ op.drop_column("users", "two_factor_allowed")
+
+
+def downgrade():
+ op.add_column(
+ "users",
+ sa.Column(
+ "two_factor_allowed",
+ sa.BOOLEAN(),
+ server_default=sa.text("false"),
+ autoincrement=False,
+ nullable=False,
+ ),
+ )
| diff --git a/tests/common/db/accounts.py b/tests/common/db/accounts.py
--- a/tests/common/db/accounts.py
+++ b/tests/common/db/accounts.py
@@ -34,7 +34,6 @@ class Meta:
datetime.datetime(2005, 1, 1), datetime.datetime(2010, 1, 1)
)
last_login = factory.fuzzy.FuzzyNaiveDateTime(datetime.datetime(2011, 1, 1))
- two_factor_allowed = True
class EmailFactory(WarehouseFactory):
diff --git a/tests/unit/accounts/test_models.py b/tests/unit/accounts/test_models.py
--- a/tests/unit/accounts/test_models.py
+++ b/tests/unit/accounts/test_models.py
@@ -12,7 +12,7 @@
import pytest
-from warehouse.accounts.models import User, UserFactory
+from warehouse.accounts.models import Email, User, UserFactory
from ...common.db.accounts import (
EmailFactory as DBEmailFactory,
@@ -37,6 +37,26 @@ def test_travel_cant_find(self, db_request):
with pytest.raises(KeyError):
root[user.username + "invalid"]
+ @pytest.mark.parametrize(
+ ("email", "verified", "allowed"),
+ [
+ ("[email protected]", True, True),
+ (None, False, False),
+ ("[email protected]", False, False),
+ ],
+ )
+ def test_two_factor_provisioning_allowed(
+ self, db_session, email, verified, allowed
+ ):
+ user = DBUserFactory.create()
+
+ if email:
+ e = Email(email=email, user=user, primary=True, verified=verified)
+ db_session.add(e)
+ db_session.flush()
+
+ assert user.two_factor_provisioning_allowed == allowed
+
class TestUser:
def test_get_primary_email_when_no_emails(self, db_session):
diff --git a/tests/unit/accounts/test_services.py b/tests/unit/accounts/test_services.py
--- a/tests/unit/accounts/test_services.py
+++ b/tests/unit/accounts/test_services.py
@@ -347,6 +347,7 @@ def test_updating_password_undisables(self, user_service):
def test_has_two_factor(self, user_service):
user = UserFactory.create()
assert not user_service.has_two_factor(user.id)
+
user_service.update_user(user.id, totp_secret=b"foobar")
assert user_service.has_two_factor(user.id)
@@ -357,6 +358,7 @@ def test_check_totp_value(self, user_service, monkeypatch, valid):
user = UserFactory.create()
user_service.update_user(user.id, totp_secret=b"foobar")
+ user_service.add_email(user.id, "[email protected]", primary=True, verified=True)
assert user_service.check_totp_value(user.id, b"123456") == valid
diff --git a/tests/unit/manage/test_views.py b/tests/unit/manage/test_views.py
--- a/tests/unit/manage/test_views.py
+++ b/tests/unit/manage/test_views.py
@@ -691,6 +691,7 @@ def test_generate_totp_qr(self, monkeypatch):
username="foobar",
email=pretend.stub(),
name=pretend.stub(),
+ two_factor_provisioning_allowed=True,
),
registry=pretend.stub(settings={"site.name": "not_a_real_site_name"}),
)
@@ -713,6 +714,7 @@ def test_generate_totp_qr_already_provisioned(self, monkeypatch):
username="foobar",
email=pretend.stub(),
name=pretend.stub(),
+ two_factor_provisioning_allowed=True,
),
)
@@ -722,6 +724,25 @@ def test_generate_totp_qr_already_provisioned(self, monkeypatch):
assert isinstance(result, Response)
assert result.status_code == 403
+ def test_generate_totp_qr_two_factor_not_allowed(self):
+ user_service = pretend.stub()
+ request = pretend.stub(
+ session=pretend.stub(flash=pretend.call_recorder(lambda *a, **kw: None)),
+ find_service=lambda interface, **kw: {IUserService: user_service}[
+ interface
+ ],
+ user=pretend.stub(two_factor_provisioning_allowed=False),
+ )
+
+ view = views.ProvisionTOTPViews(request)
+ result = view.generate_totp_qr()
+
+ assert isinstance(result, Response)
+ assert result.status_code == 403
+ assert request.session.flash.calls == [
+ pretend.call("Modifying 2FA requires a verified email.", queue="error")
+ ]
+
def test_totp_provision(self, monkeypatch):
user_service = pretend.stub(get_totp_secret=lambda id: None)
request = pretend.stub(
@@ -737,6 +758,7 @@ def test_totp_provision(self, monkeypatch):
username=pretend.stub(),
email=pretend.stub(),
name=pretend.stub(),
+ two_factor_provisioning_allowed=True,
),
registry=pretend.stub(settings={"site.name": "not_a_real_site_name"}),
)
@@ -774,6 +796,7 @@ def test_totp_provision_already_provisioned(self, monkeypatch):
username=pretend.stub(),
email=pretend.stub(),
name=pretend.stub(),
+ two_factor_provisioning_allowed=True,
),
route_path=lambda *a, **kw: "/foo/bar/",
)
@@ -787,6 +810,25 @@ def test_totp_provision_already_provisioned(self, monkeypatch):
pretend.call("TOTP already provisioned.", queue="error")
]
+ def test_totp_provision_two_factor_not_allowed(self):
+ user_service = pretend.stub()
+ request = pretend.stub(
+ session=pretend.stub(flash=pretend.call_recorder(lambda *a, **kw: None)),
+ find_service=lambda interface, **kw: {IUserService: user_service}[
+ interface
+ ],
+ user=pretend.stub(two_factor_provisioning_allowed=False),
+ )
+
+ view = views.ProvisionTOTPViews(request)
+ result = view.totp_provision()
+
+ assert isinstance(result, Response)
+ assert result.status_code == 403
+ assert request.session.flash.calls == [
+ pretend.call("Modifying 2FA requires a verified email.", queue="error")
+ ]
+
def test_validate_totp_provision(self, monkeypatch):
user_service = pretend.stub(
get_totp_secret=lambda id: None,
@@ -807,6 +849,7 @@ def test_validate_totp_provision(self, monkeypatch):
username=pretend.stub(),
email=pretend.stub(),
name=pretend.stub(),
+ two_factor_provisioning_allowed=True,
),
route_path=lambda *a, **kw: "/foo/bar/",
)
@@ -843,6 +886,7 @@ def test_validate_totp_provision_already_provisioned(self, monkeypatch):
username=pretend.stub(),
email=pretend.stub(),
name=pretend.stub(),
+ two_factor_provisioning_allowed=True,
),
route_path=pretend.call_recorder(lambda *a, **kw: "/foo/bar"),
)
@@ -873,6 +917,7 @@ def test_validate_totp_provision_invalid_form(self, monkeypatch):
username=pretend.stub(),
email=pretend.stub(),
name=pretend.stub(),
+ two_factor_provisioning_allowed=True,
),
registry=pretend.stub(settings={"site.name": "not_a_real_site_name"}),
)
@@ -899,6 +944,25 @@ def test_validate_totp_provision_invalid_form(self, monkeypatch):
"provision_totp_uri": "not_a_real_uri",
}
+ def test_validate_totp_provision_two_factor_not_allowed(self):
+ user_service = pretend.stub()
+ request = pretend.stub(
+ session=pretend.stub(flash=pretend.call_recorder(lambda *a, **kw: None)),
+ find_service=lambda interface, **kw: {IUserService: user_service}[
+ interface
+ ],
+ user=pretend.stub(two_factor_provisioning_allowed=False),
+ )
+
+ view = views.ProvisionTOTPViews(request)
+ result = view.validate_totp_provision()
+
+ assert isinstance(result, Response)
+ assert result.status_code == 403
+ assert request.session.flash.calls == [
+ pretend.call("Modifying 2FA requires a verified email.", queue="error")
+ ]
+
def test_delete_totp(self, monkeypatch, db_request):
user_service = pretend.stub(
get_totp_secret=lambda id: b"secret",
@@ -914,6 +978,7 @@ def test_delete_totp(self, monkeypatch, db_request):
email=pretend.stub(),
name=pretend.stub(),
totp_secret=b"secret",
+ two_factor_provisioning_allowed=True,
),
route_path=lambda *a, **kw: "/foo/bar/",
)
@@ -948,6 +1013,7 @@ def test_delete_totp_bad_username(self, monkeypatch, db_request):
username=pretend.stub(),
email=pretend.stub(),
name=pretend.stub(),
+ two_factor_provisioning_allowed=True,
),
route_path=lambda *a, **kw: "/foo/bar/",
)
@@ -980,6 +1046,7 @@ def test_delete_totp_not_provisioned(self, monkeypatch, db_request):
username=pretend.stub(),
email=pretend.stub(),
name=pretend.stub(),
+ two_factor_provisioning_allowed=True,
),
route_path=lambda *a, **kw: "/foo/bar/",
)
@@ -998,6 +1065,25 @@ def test_delete_totp_not_provisioned(self, monkeypatch, db_request):
assert isinstance(result, HTTPSeeOther)
assert result.headers["Location"] == "/foo/bar/"
+ def test_delete_totp_two_factor_not_allowed(self):
+ user_service = pretend.stub()
+ request = pretend.stub(
+ session=pretend.stub(flash=pretend.call_recorder(lambda *a, **kw: None)),
+ find_service=lambda interface, **kw: {IUserService: user_service}[
+ interface
+ ],
+ user=pretend.stub(two_factor_provisioning_allowed=False),
+ )
+
+ view = views.ProvisionTOTPViews(request)
+ result = view.delete_totp()
+
+ assert isinstance(result, Response)
+ assert result.status_code == 403
+ assert request.session.flash.calls == [
+ pretend.call("Modifying 2FA requires a verified email.", queue="error")
+ ]
+
class TestManageProjects:
def test_manage_projects(self, db_request):
| Require verified email before allowing user to enable 2FA
**What's the problem this feature will solve?**
Sometimes people enable two-factor auth and then run into some kind of problem like losing access to their provisioned TOTP-generating app. We want to make it easier for PyPI admins to check the legitimacy of users' requests for account recovery in such circumstances.
**Describe the solution you'd like**
Prohibit users from enabling any 2FA until the user has verified at least one email address on their account.
**Additional context**
(followup to #5661, filed on @di's behalf)
| Working on this now. | 2019-05-20T21:37:08Z | [] | [] |
pypi/warehouse | 5,942 | pypi__warehouse-5942 | [
"5940"
] | e3bb425c7ea47ccd2773b2840f32afb5864e7762 | diff --git a/warehouse/accounts/services.py b/warehouse/accounts/services.py
--- a/warehouse/accounts/services.py
+++ b/warehouse/accounts/services.py
@@ -257,6 +257,28 @@ def check_totp_value(self, user_id, totp_value, *, tags=None):
tags = tags if tags is not None else []
self._metrics.increment("warehouse.authentication.two_factor.start", tags=tags)
+ # The very first thing we want to do is check to see if we've hit our
+ # global rate limit or not, assuming that we've been configured with a
+ # global rate limiter anyways.
+ if not self.ratelimiters["global"].test():
+ logger.warning("Global failed login threshold reached.")
+ self._metrics.increment(
+ "warehouse.authentication.two_factor.ratelimited",
+ tags=tags + ["ratelimiter:global"],
+ )
+ raise TooManyFailedLogins(resets_in=self.ratelimiters["global"].resets_in())
+
+ # Now, check to make sure that we haven't hitten a rate limit on a
+ # per user basis.
+ if not self.ratelimiters["user"].test(user_id):
+ self._metrics.increment(
+ "warehouse.authentication.two_factor.ratelimited",
+ tags=tags + ["ratelimiter:user"],
+ )
+ raise TooManyFailedLogins(
+ resets_in=self.ratelimiters["user"].resets_in(user_id)
+ )
+
totp_secret = self.get_totp_secret(user_id)
if totp_secret is None:
| diff --git a/tests/unit/accounts/test_services.py b/tests/unit/accounts/test_services.py
--- a/tests/unit/accounts/test_services.py
+++ b/tests/unit/accounts/test_services.py
@@ -366,6 +366,46 @@ def test_check_totp_value_no_secret(self, user_service):
user = UserFactory.create()
assert not user_service.check_totp_value(user.id, b"123456")
+ def test_check_totp_global_rate_limited(self, user_service, metrics):
+ resets = pretend.stub()
+ limiter = pretend.stub(test=lambda: False, resets_in=lambda: resets)
+ user_service.ratelimiters["global"] = limiter
+
+ with pytest.raises(TooManyFailedLogins) as excinfo:
+ user_service.check_totp_value(uuid.uuid4(), b"123456", tags=["foo"])
+
+ assert excinfo.value.resets_in is resets
+ assert metrics.increment.calls == [
+ pretend.call("warehouse.authentication.two_factor.start", tags=["foo"]),
+ pretend.call(
+ "warehouse.authentication.two_factor.ratelimited",
+ tags=["foo", "ratelimiter:global"],
+ ),
+ ]
+
+ def test_check_totp_value_user_rate_limited(self, user_service, metrics):
+ user = UserFactory.create()
+ resets = pretend.stub()
+ limiter = pretend.stub(
+ test=pretend.call_recorder(lambda uid: False),
+ resets_in=pretend.call_recorder(lambda uid: resets),
+ )
+ user_service.ratelimiters["user"] = limiter
+
+ with pytest.raises(TooManyFailedLogins) as excinfo:
+ user_service.check_totp_value(user.id, b"123456")
+
+ assert excinfo.value.resets_in is resets
+ assert limiter.test.calls == [pretend.call(user.id)]
+ assert limiter.resets_in.calls == [pretend.call(user.id)]
+ assert metrics.increment.calls == [
+ pretend.call("warehouse.authentication.two_factor.start", tags=[]),
+ pretend.call(
+ "warehouse.authentication.two_factor.ratelimited",
+ tags=["ratelimiter:user"],
+ ),
+ ]
+
class TestTokenService:
def test_verify_service(self):
| 2FA validation should be rate limited, in addition to current window
**What's the problem this feature will solve?**
Right now, we use a signed parameter with a 5-minute window to ensure authority/integrity during 2FA authentication. As a result, any user who successfully enters a password but fails to enter a correct TOTP code (or WebAuthn attestation, soon) within 5 minutes will be kicked back to the login page and required to re-enter the password.
The above provides sufficient brute-force protection, but it'd be nice to additionally leverage Warehouse's existing rate-limiters to prevent an attacker from wasting resources by hammering TOTP verification within each 5 minute window.
**Describe the solution you'd like**
An additional user-scoped ratelimiter on `check_totp_value` (and eventually WebAuthn) with similar semantics to the user-scope ratelimiter on `check_password`.
| 2019-05-31T19:02:00Z | [] | [] |