branch_name
stringclasses 15
values | target
stringlengths 26
10.3M
| directory_id
stringlengths 40
40
| languages
sequencelengths 1
9
| num_files
int64 1
1.47k
| repo_language
stringclasses 34
values | repo_name
stringlengths 6
91
| revision_id
stringlengths 40
40
| snapshot_id
stringlengths 40
40
| input
stringclasses 1
value |
---|---|---|---|---|---|---|---|---|---|
refs/heads/master | <file_sep>import React from 'react';
import PropTypes from 'prop-types';
import { withStyles } from '@material-ui/core/styles';
import Button from '@material-ui/core/Button';
import Tooltip from '@material-ui/core/Tooltip';
// button to trigger search in browser
class SearchButton extends React.Component {
state = {};
buttonLabel = (this.props.variant === 'search')? 'Suchen' : 'gesamten Korpus herunterladen';
buttonVariant = (this.props.variant === 'search')? 'contained' : 'text';
// display tip on hover
searchTip = 'Alle Texte abfragen, die den eingegebenen Kriterien entsprechen';
getAllTip = 'Alle verfügbaren Texte abfragen';
buttonTips = this.props.variant === 'search'? this.searchTip : this.getAllTip;
render() {
const { classes } = this.props;
return(
<div className={classes.root}>
<Tooltip title={this.buttonTips} placement={'bottom-start'}>
<Button
color={'primary'}
variant={this.buttonVariant}
disabled={this.props.getLoading()}
onClick={this.props.handleSubmit}
>
{this.buttonLabel}
</Button>
</Tooltip>
</div>
)
}
}
SearchButton.propTypes = {
classes: PropTypes.object.isRequired,
variant: PropTypes.string,
getLoading: PropTypes.func.isRequired,
handleSubmit: PropTypes.func.isRequired,
};
const styles = theme => ({
root:{
margin: theme.spacing.unit,
}
});
export default withStyles(styles)(SearchButton);<file_sep>import React from 'react';
import PropTypes from 'prop-types';
import {withStyles} from '@material-ui/core/styles';
import Typography from '@material-ui/core/Typography';
import InfoCard from '../InfoCard';
import NotificationContext from '../notifications/NotificationContext';
import Link from '@material-ui/core/Link';
class Downloads extends React.Component {
state = {
filenames: [],
};
handleChange = name => event => {
this.setState({
[name]: event.target.value,
});
};
componentDidMount() {
this.requestStatus();
};
logout = () => {
this.handleStateChange('loggedIn', false);
this.handleStateChange('isAdmin', false);
};
requestFilenames = () => {
fetch("/backend/lib/functions.php", {
method: 'POST',
credentials: 'same-origin', // allow cookies -> session management
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({getFiles: true})
})
.then(res => {
if(res.ok) {
res.json().then(data => {
if (data && data.status === "success") {
this.context.handleNotificationChange(true, 'Die Dateien wurden erfolgreich abgerufen.', 'getFiles', 'success');
this.setState({filenames: data.files})
} else {
this.context.handleNotificationChange(true, 'Die Dateien konnten nicht vom Server geladen werden.', 'getFiles', 'error');
}
})
} else {
this.context.handleNotificationChange(true, 'Die Dateien konnten nicht vom Server geladen werden.', 'getFiles', 'error');
}
})
.catch(error => {
this.context.handleNotificationChange(true, 'Die Dateien konnten nicht vom Server geladen werden.', 'getFiles', 'error');
}
);
};
requestStatus = () => {
// check if session is still valid otherwise logout user
fetch("/backend/lib/sessionManagement.php", {
method: 'POST',
credentials: 'same-origin', // allow cookies -> session management
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({
loginStatus: true,
loginID: this.props.sessionID
})
}).then(response => {
if(response.ok) {
response.json().then(data => {
if(!data || data.status === 'error') {
this.context.handleNotificationChange(true, 'Ihre Sitzung ist abgelaufen.', 'sessionCheck', 'error');
this.props.handleAppChange('loggedIn', false);
this.props.handleAppChange('isAdmin', false);
} else {
this.requestFilenames();
}
});
} else {
this.context.handleNotificationChange(true, 'Ihre Sitzung ist abgelaufen.', 'sessionCheck', 'error');
this.logout();
}
}
).catch(error => {
this.context.handleNotificationChange(true, 'Ihre Sitzung ist abgelaufen.', 'sessionCheck', 'error', 404);
this.logout();
}
);
};
render() {
const {classes} = this.props;
const {filenames} = this.state;
return (
<div className={classes.root}>
<InfoCard
message='Auf dieser Seite können Sie die Nutzerdokumentation mit Hinweisen zur Seite und
Skripte zur weiteren Verarbeitung der Texte herunterladen.'
/>
<div className={classes.textContainer}>
<Typography variant={'h5'} color={'primary'}>Hinweise für Nutzer*innen</Typography>
<ul>
{filenames
.filter(name => name.includes('.pdf'))
.map(name => <li><Link key={name} href={'/backend/scripts/' + name} download>{name}</Link></li>)}
</ul>
<Typography variant={'h5'} color={'primary'}>Skripte</Typography>
<ul>
{filenames.map(name => {
if(!name.includes('.pdf')) {
return <li><Link key={name} href={'/backend/scripts/' + name} download>{name}</Link></li>
}
}
)}
</ul>
</div>
</div>
)
}
}
Downloads.propTypes = {
classes: PropTypes.object.isRequired,
handleAppChange: PropTypes.func.isRequired,
sessionID: PropTypes.any.isRequired,
};
const styles = theme => ({
root:{
margin: theme.spacing.unit * 3,
marginTop: theme.spacing.unit * 4,
},
textContainer: {
minWidth: 500,
maxWidth: 1000,
marginLeft: theme.spacing.unit,
}
});
Downloads.contextType = NotificationContext;
export default withStyles(styles)(Downloads);<file_sep>import React from 'react';
import PropTypes from 'prop-types';
import {withStyles} from '@material-ui/core/styles';
import Paper from "@material-ui/core/Paper/Paper";
import Typography from '@material-ui/core/Typography';
import Button from '@material-ui/core/Button';
import TextField from '@material-ui/core/TextField';
import InfoIcon from '@material-ui/icons/Info';
import CircularProgress from '@material-ui/core/CircularProgress';
import NotificationContext from "../../notifications/NotificationContext";
class Login extends React.Component {
state = {
username: '',
password: '',
loading: false,
};
onInputChange = (event) => {
this.setState({[event.target.name]: event.target.value});
};
onKeyPress = (event) => {
if(event.key === 'Enter') {
this.requestLogin();
event.preventDefault();
}
};
// send request to server + change app state or display error
requestLogin = () => {
if(this.state.username.length < 1 || this.state.password.length < 1) {
this.context.handleNotificationChange(true, 'Bitte geben Sie einen Username und ein Passwort ein.', 'login', 'error');
return;
}
this.setState({
loading: true,
error: false,
});
fetch('/backend/lib/sessionManagement.php',{
method: 'POST',
credentials: 'same-origin', // allow cookies -> session management
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({
login: true,
username: this.state.username,
password: <PASSWORD>,
}),
})
.then(response => {
this.setState({statusCode: response.status});
if (response.ok) {
response.json().then(data => {
if(data && data.status === 'success' && typeof data.isadmin !== 'undefined') {
this.props.handleAppStateChange('loggedIn', true);
this.props.handleAppStateChange('isAdmin', data.isadmin !== 0);
this.props.handleAppStateChange('sessionID', data.id);
this.context.handleNotificationChange(true, 'Login erfolgreich.', 'login', 'success');
} else {
// server error
this.context.handleNotificationChange(true, 'Es ist ein Fehler beim Login aufgetreten.', 'login', 'error', data.error);
}
});
} else {
this.setState({
loginError: true,
errorMessage: response.statusText,
});
this.context.handleNotificationChange(true, response.statusText, 'login', 'error', response.statusCode);
}
})
.catch(error => {
this.setState({
loginError: true,
errorMessage: error.message,
});
this.context.handleNotificationChange(true, error.message, 'login', 'error', 404);
});
this.setState({loading: false});
};
render() {
const {classes } = this.props;
const { username, password, loading } = this.state;
return (
<div className={classes.root}>
<Paper className={classes.infoBox}>
<InfoIcon color={"primary"} className={classes.infoIcon}/>
<Typography color={"primary"}>
Falls Sie sich registrieren möchten, Ihr Passwort vergessen haben
oder Ihr Nutzerkonto löschen möchten wenden Sie sich bitte an den/die Administrator/in.
</Typography>
</Paper>
<Paper className={classes.loginContainer}>
<div className={classes.formContainer}>
<Typography variant={'h6'} color={'primary'}>Bitte melden Sie sich an</Typography>
<TextField
disabled={loading}
autoFocus={true}
className={classes.textField}
label={'Benutzername'}
type={'text'}
name={'username'}
value={username}
onChange={this.onInputChange}
onKeyPress={this.onKeyPress}
/>
<TextField
className={classes.textField}
disabled={loading}
label={'Passwort'}
type={'password'}
name={'password'}
value={<PASSWORD>}
onChange={this.onInputChange}
onKeyPress={this.onKeyPress}
/>
<div className={classes.flexContainer}>
<Button
disabled={loading}
size="small"
color="primary"
variant={"contained"}
className={classes.button}
onClick={this.requestLogin}
>
Anmelden
</Button>
{loading && <CircularProgress className={classes.loadingAnimation} size={30}/>}
</div>
</div>
</Paper>
</div>
)
}
}
Login.propTypes = {
classes: PropTypes.object.isRequired,
url: PropTypes.string,
handleAppStateChange: PropTypes.func,
};
Login.contextType = NotificationContext;
const styles = theme => ({
root: {
marginTop: theme.spacing.unit * 4
},
loginContainer:{
width: 400,
padding: theme.spacing.unit * 6,
margin: 'auto',
},
formContainer:{
display: 'flex',
flexDirection: 'column',
},
textField: {
marginTop: theme.spacing.unit * 2,
},
button:{
marginTop: theme.spacing.unit * 5,
marginRight: theme.spacing.unit * 2,
width: 100,
},
infoBox:{
width: 400 + theme.spacing.unit * 6,
padding: theme.spacing.unit*2,
paddingRight: theme.spacing.unit * 4,
display: 'flex',
margin: 'auto',
marginBottom: theme.spacing.unit * 3,
},
infoIcon: {
marginRight: theme.spacing.unit,
},
flexContainer:{
display: 'flex',
alignItems: 'flex-end',
},
loginErrorMessage:{
marginLeft: -theme.spacing.unit,
marginTop: theme.spacing.unit * 3,
width: theme.spacing.unit * 52,
},
});
export default withStyles(styles)(Login);<file_sep>import React from 'react';
import PropTypes from 'prop-types';
import { withStyles } from '@material-ui/core/styles';
import Paper from "@material-ui/core/Paper/Paper";
import InfoButton from '@material-ui/icons/Info';
import CircularProgress from '@material-ui/core/CircularProgress';
import Typography from '@material-ui/core/Typography';
import NotificationContext from '../../notifications/NotificationContext';
import AuthorInput from "./searchcard/AuthorInput";
import GenreSelection from "./searchcard/GenreSelection";
import ContainsInput from "./searchcard/ContainsInput";
import TimeInput from "./searchcard/TimeInput";
import SearchCard from './searchcard/SearchCard';
import AddSearchCardButton from './buttons/AddSearchCardButton';
import SelectFormat from './SelectFormat';
import SearchButton from './buttons/SearchButton';
import Results from './results/Results';
import shortid from 'shortid';
// MUI variant of input fields inside browser
const inputVariant = 'standard';
// max. number of search cards that can be added by user
const maxCards = 4;
class Browser extends React.PureComponent {
initialSearchCardObject = {
id: shortid.generate(),
authors: [],
genres: [],
keywords: '',
timeFrom: '',
timeTo: '',
};
state = {
cardList: [JSON.parse(JSON.stringify(this.initialSearchCardObject))],
selectedFormats: {checkedTXT: false, checkedJSON: false},
loading: false,
responseFiles: '',
hits: 0,
responseIn: false,
error: false,
errorMessage: 'no error',
timeError: false,
keywordError: false,
formatError: true,
searchID: 0,
getAll: false,
};
getCardIndex = id => {
return this.state.cardList.findIndex(card => card.id === id);
};
getLoading = () => {
return this.state.loading;
};
handleChange = (name, value) => {
this.setState({
[name]: value,
});
};
// add new search card
onAddSearchCard = inputValues => {
if(this.state && this.state.cardList.length >= maxCards ) {
this.context.handleNotificationChange(true, `Ihre Anfrage enthält bereits ${maxCards} Teilsuchen.`, 'addCard', 'error');
return;
}
let paramsPassed = (inputValues instanceof Object && 'authors' in inputValues);
inputValues = paramsPassed?
JSON.parse(JSON.stringify(inputValues)) : JSON.parse(JSON.stringify(this.initialSearchCardObject));
inputValues.id = shortid.generate();
this.setState(state => ({
cardList: [...state.cardList, inputValues]
}), () => {
if(this.state.cardList.length === maxCards) {
this.context.handleNotificationChange(true, `Sie können max. ${maxCards} Teilsuchen erstellen.`, 'addCard', 'warning');
}
});
};
// duplicate existing search card with content
onDuplicateSearchCard = id => {
this.onAddSearchCard(this.state.cardList.find(card => card.id === id));
};
updateSearchCardContent = (id, prop, value) => {
if(this.state && this.state.cardList) {
let newCardList = JSON.parse(JSON.stringify(this.state.cardList));
newCardList.find(card => card.id === id)[prop] = JSON.parse(JSON.stringify(value));
this.setState({cardList: newCardList });
}
};
deleteSearchCard = id => {
// last remaining card should not be deleted => warning
if(this.state.cardList.length === 1) {
this.context.handleNotificationChange(true, 'Ihre Anfrage muss min. 1 Teil-Suche enthalten.', 'deleteCard', 'warning');
return;
}
this.setState(state => ({
cardList: state.cardList.filter( card => (card.id !== id))
}));
};
// update list of selected formats for results (txt, json)
updateFormat = (formatProp, newValue) => {
let newSelectedFormats = JSON.parse(JSON.stringify(this.state.selectedFormats));
newSelectedFormats[formatProp] = JSON.parse(JSON.stringify(newValue));
this.setState({selectedFormats: newSelectedFormats});
};
// see if user is still logged in
requestStatus = func => {
// check if session is still valid otherwise logout user
fetch("/backend/lib/sessionManagement.php", {
method: 'POST',
credentials: 'same-origin', // allow cookies -> session management
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({
loginStatus: true,
loginID: this.props.sessionID
})
}).then(response => {
if(response.ok) {
response.json().then(data => {
if(!data || data.status === 'error') {
this.context.handleNotificationChange(true, 'Ihre Sitzung ist abgelaufen.', 'sessionCheck', 'error');
this.props.handleAppChange('loggedIn', false);
this.props.handleAppChange('isAdmin', false);
} else {
func();
}
});
} else {
this.context.handleNotificationChange(true, 'Ihre Sitzung ist abgelaufen.', 'sessionCheck', 'error');
this.logout();
}
}
).catch(error => {
this.context.handleNotificationChange(true, 'Ihre Sitzung ist abgelaufen.', 'sessionCheck', 'error', 404);
this.logout();
}
);
};
// submit search with or without criteria
handleSubmit = (getAll) => {
// check for errors before sending request
if ((!getAll && (this.state.timeError || this.state.keywordError)) || this.state.formatError) {
this.setState({
error: true,
errorMessage: 'Sie können keine Anfrage mit fehlenden/falschen Eingaben abschicken',
});
this.context.handleNotificationChange(true, 'Sie können keine Anfrage mit fehlenden/falschen Eingaben abschicken', 'login', 'error');
return;
}
const payload = getAll ? {getAll: true} : {cards: this.state.cardList};
// start loading animation, disable forms
this.setState({
loading: true,
error: false,
responseIn: false,
responseFiles: [],
getAll: false,
});
// request data + handle response
fetch("/backend/lib/functions.php", {
method: 'POST',
credentials: 'same-origin', // allow cookies -> session management
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify(payload)
})
.then(response => {
if (response.ok) {
response.json().then(data => {
if (data && data.status && data.status === 'success') {
// search succeeded (there are results)
this.setState({
responseFiles: data.filenames, // array of filenames separated by comma
hits: data.hits, // number
responseIn: true,
searchID: shortid.generate(),
}, () => {
this.setState({loading: false});
});
} else {
// server error / search not possible on server
this.setState({
errorMessage: 'Es ist ein Fehler auf dem Server aufgetreten.',
error: true
},() => {
this.setState({loading: false});
});
this.context.handleNotificationChange(true, 'Es ist ein Fehler auf dem Server aufgetreten. Die Anfrage wurde abgebrochen.', 'search', 'error');
}
});
} else {
// other problem
this.setState({
errorMessage: response.statusText,
error: true
},() => {
this.setState({loading: false});
});
this.context.handleNotificationChange(true, response.statusText, 'search', 'error', response.statusCode)
}
})
.catch(error => {
this.setState({
errorMessage: error.message,
error: true
},() => {
this.setState({loading: false});
});
this.context.handleNotificationChange(true, error.message, 'search', 'error', 404)
});
//this.setState({loading: false});
};
// do search with criteria entered by user
handleSearchCriteria = () => {
this.requestStatus(() => this.handleSubmit(false));
};
// do search not regarding criteria that might have been entered by user
handleGetAll = () => {
this.setState({responseIn: true, getAll: true, searchID: shortid.generate()});
};
render() {
const { classes, authorsList, minYear, maxYear, genres, handleAppChange, sessionID } = this.props;
const { cardList, selectedFormats, responseFiles, responseIn, loading, hits, searchID, getAll} = this.state;
const renderResponseData = () => {
let formats = selectedFormats.checkedJSON? ['json'] : [];
if(selectedFormats.checkedTXT) formats.push('txt');
return <Results
filenames={responseFiles}
number={hits}
formats={formats}
sessionID={sessionID}
searchID={searchID}
getAll={getAll}
handleAppChange={handleAppChange}
/>;
};
return(
<div className={classes.root}>
<Paper className={classes.infoBox}>
<InfoButton color={"primary"} className={classes.infoIcon}/>
<Typography color={"primary"}>
Schränken Sie die Suche ein, indem Sie eines oder mehrere Kriterien innerhalb einer Teil-Suche (=Kasten) eingeben.
Teil-Suchen werden zu einer Such-Anfrage kombiniert, sodass Sie mehr Texte mit einer Suche erhalten können.
</Typography>
</Paper>
<form onSubmit={this.handleSubmit.bind(this)}>
<div className={classes.cardContainer}>
{cardList.map((card, index, cardList) => (
<SearchCard
key={card.id} // not passed to component by react! => use id instead
id={card.id}
getIndex={this.getCardIndex}
getDisabled={this.getLoading}
onDuplicate={this.onDuplicateSearchCard}
onDelete={this.deleteSearchCard}
>
<AuthorInput
key={card.id + '-authors'}
cardId={card.id}
variant={inputVariant}
initialValues={card.authors}
authorsList={authorsList}
autofocus={(index === cardList.length-1)}
disabled={this.getLoading()}
onInputChange={this.updateSearchCardContent}
/>
<GenreSelection
key={card.id + '-genres'}
cardId={card.id}
genres={genres}
variant={inputVariant}
initialValues={card.genres}
disabled={this.getLoading()}
onInputChange={this.updateSearchCardContent}
/>
<ContainsInput
key={card.id + '-keywords'}
cardId={card.id}
variant={inputVariant}
initialValue={card.keywords}
disabled={this.getLoading()}
onInputChange={this.updateSearchCardContent}
handleBrowserChange={this.handleChange}
/>
<TimeInput
key={card.id + '-time'}
cardId={card.id}
variant={inputVariant}
initialTimeFrom={card.timeFrom}
initialTimeTo={card.timeTo}
disabled={this.getLoading()}
onInputChange={this.updateSearchCardContent}
minYear={minYear}
maxYear={maxYear}
handleBrowserChange={this.handleChange}
/>
</SearchCard>
))}
</div>
<AddSearchCardButton action={this.onAddSearchCard} getDisabled={this.getLoading}/>
<div className={classes.flexContainer}>
<SelectFormat
initialValues={selectedFormats}
getDisabled={this.getLoading}
onChange={this.updateFormat}
handleBrowserChange={this.handleChange}
/>
</div>
<div className={classes.flexContainer}>
<SearchButton
type={'submit'} // form gets submitted when clicking on this button
variant={'search'}
getLoading={this.getLoading}
handleSubmit={this.handleSearchCriteria}
/>
<SearchButton
getLoading={this.getLoading}
handleSubmit={this.handleGetAll}
/>
</div>
</form>
<div className={classes.flexContainer}>
{loading && <CircularProgress className={classes.loadingAnimation} />}
{responseIn && renderResponseData()}
</div>
</div>
);
}
}
Browser.propTypes = {
classes: PropTypes.object.isRequired,
authorsList: PropTypes.arrayOf(PropTypes.string).isRequired,
minYear: PropTypes.string.isRequired,
maxYear: PropTypes.string.isRequired,
genres: PropTypes.arrayOf(PropTypes.string),
handleAppChange: PropTypes.func.isRequired,
sessionID: PropTypes.any.isRequired,
};
Browser.contextType = NotificationContext;
const styles = theme => ({
root:{
paddingLeft: theme.spacing.unit * 3,
paddingBottom: theme.spacing.unit * 3,
marginTop: theme.spacing.unit * 4,
},
infoBox:{
minWidth: 400,
maxWidth: 800 + theme.spacing.unit*7,
padding: 20,
display: 'flex',
marginLeft: theme.spacing.unit,
marginBottom: theme.spacing.unit * 3,
},
infoIcon: {
marginRight: theme.spacing.unit,
},
cardContainer:{
display: 'flex',
flexFlow: 'row wrap',
},
flexContainer:{
display: 'flex',
marginTop: theme.spacing.unit * 2,
},
cardWarning:{
margin: theme.spacing.unit,
},
loadingAnimation:{
marginLeft: theme.spacing.unit,
},
});
export default withStyles(styles)(Browser);<file_sep>import React from 'react';
import PropTypes from 'prop-types';
import {withStyles} from '@material-ui/core/styles';
import {Switch, Route} from 'react-router-dom';
import AdminNav from './AdminNav';
import TextManagement from './tabs/TextManagement';
import ServerManagement from './tabs/ServerManagement';
import UserManagement from './tabs/UserManagement';
import MissingPage from '../MissingPage';
import NotificationContext from '../../notifications/NotificationContext';
// routing for Admin page
class Admin extends React.Component {
state = {};
handleChange = name => event => {
this.setState({
[name]: event.target.value,
});
};
requestStatus = func => {
// check if session is still valid otherwise logout user
fetch("/backend/lib/sessionManagement.php", {
method: 'POST',
credentials: 'same-origin', // allow cookies -> session management
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({
loginStatus: true,
loginID: this.props.sessionID
})
}).then(response => {
if(response.ok) {
response.json().then(data => {
if(!data || data.status === 'error') {
this.context.handleNotificationChange(true, 'Ihre Sitzung ist abgelaufen.', 'sessionCheck', 'error');
this.props.handleAppChange('loggedIn', false);
this.props.handleAppChange('isAdmin', false);
} else {
func();
}
});
} else {
this.context.handleNotificationChange(true, 'Ihre Sitzung ist abgelaufen.', 'sessionCheck', 'error');
this.logout();
}
}
).catch(error => {
this.context.handleNotificationChange(true, 'Ihre Sitzung ist abgelaufen.', 'sessionCheck', 'error', 404);
this.logout();
}
);
};
render() {
const {classes, requestNewAuthors, requestNewLog} = this.props;
return (
<div className={classes.root}>
<AdminNav/>
<div className={classes.pageContainer}>
<Switch>
<Route path='/admin/users' render={() => <UserManagement requestStatus={this.requestStatus} userStatus={this.requestStatus}/>} />
<Route path='/admin/server' render={() => <ServerManagement requestStatus={this.requestStatus} userStatus={this.requestStatus}/>} />
<Route path='/admin' render={() =>
<TextManagement
requestNewAuthors={requestNewAuthors}
requestNewLog={requestNewLog}
requestStatus={this.requestStatus}
/>}
/>
<Route exact path='/admin/:other' render={() => <MissingPage/>} />
</Switch>
</div>
</div>
)
}
}
Admin.propTypes = {
classes: PropTypes.object.isRequired,
requestNewAuthors: PropTypes.func.isRequired,
requestNewLog: PropTypes.func.isRequired,
handleAppChange: PropTypes.func.isRequired,
sessionID: PropTypes.any.isRequired,
};
Admin.contextType = NotificationContext;
const styles = theme => ({
root: {
marginLeft: theme.spacing.unit * 4,
marginTop: theme.spacing.unit * 4,
},
pageContainer:{
paddingLeft: 200,
}
});
export default withStyles(styles)(Admin);<file_sep>import React from "react";
// create React context to provide access to App Notification for children
const NotificationContext = React.createContext({});
export default NotificationContext;<file_sep>import { createMuiTheme } from '@material-ui/core/styles';
import indigo from '@material-ui/core/colors/indigo';
import red from '@material-ui/core/colors/red';
import grey from '@material-ui/core/colors/grey';
const theme = createMuiTheme({
palette: {
primary: {
light: indigo[300],
main: indigo[600],
dark: indigo[900],
},
secondary: {
light: red[300],
main: red[600],
dark: red[900],
},
background: grey,
},
typography: {
useNextVariants: true,
},
});
export default theme;<file_sep>import React from 'react';
import PropTypes from 'prop-types';
import { withStyles } from '@material-ui/core/styles';
import Button from '@material-ui/core/Button';
import AddIcon from '@material-ui/icons/Add';
// button to add new empty search card in browser
class AddSearchCardButton extends React.Component {
state = {
};
render() {
const { classes, action, getDisabled } = this.props;
return(
<div className={classes.root}>
<Button
color="primary"
variant="outlined"
disabled={getDisabled()}
onClick={action}>
<AddIcon/>
neue Teil-Suche hinzufügen
</Button>
</div>
)
}
}
AddSearchCardButton.propTypes = {
classes: PropTypes.object.isRequired,
action: PropTypes.func.isRequired,
getDisabled: PropTypes.func.isRequired,
};
const styles = theme => ({
root: {
margin: theme.spacing.unit,
},
});
export default withStyles(styles)(AddSearchCardButton);<file_sep>import React from 'react';
import PropTypes from 'prop-types';
import { withStyles } from '@material-ui/core/styles';
import Typography from '@material-ui/core/Typography';
// about the project, impressum
class About extends React.Component {
state = {
};
render() {
const { classes } = this.props;
return(
<div className={classes.root}>
<Typography variant={'h5'} color={'primary'} className={classes.aboutText}>Über diese Seite</Typography><br/>
<Typography className={classes.aboutText} paragraph={true}>
Diese Webseite ist das Ergebnis des Projekts “Gutenberg German Poetry Corpus” im Rahmen des Moduls “Interdisziplinäres Medienprojekt” des Studiengangs B.Sc. Medieninformatik an der Freien Universität Berlin und Technischen Universität Berlin.
Die Motivation dieses Projektes ist es, die Arbeit von beispielsweise Linguist*innen und Psycholog*innen zu erleichtern, um Erkenntnisse über die in Gedichten verwendete Sprache zu erlangen.
Das Ergebnis ist diese Webanwendung, die es ermöglicht auf die Gedichte des “Gutenberg-Korpus” (des größten deutschsprachigen Gedichts-Korpus) zuzugreifen, diese nach bestimmten Kriterien zu filtern und weitere Texte hinzuzufügen.
</Typography>
<Typography className={classes.aboutText} paragraph={true}>
Projektleiterin war Frau Dr. <NAME>, wissenschaftliche Mitarbeiterin im Fachgebiet Allgemeine und neurokognitive Psychologie an der Freien Universität Berlin. Assistiert hat <NAME>, Studentin der Medieninformatik an der TU und FU Berlin.
Am Projekt mitgewirkt haben <NAME>, <NAME>, <NAME>, <NAME> und <NAME>asiliou.
</Typography>
<Typography className={classes.aboutText}>
Favicon made by {' '}<a href="https://www.freepik.com/" title="Freepik">Freepik</a>{' '}
from <a href="https://www.flaticon.com/" title="Flaticon">www.flaticon.com</a> is licensed by{' '}
<a href="http://creativecommons.org/licenses/by/3.0/" title="Creative Commons BY 3.0" target="_blank" rel="noopener noreferrer">CC 3.0 BY</a>
</Typography>
</div>
);
}
}
About.propTypes = {
classes: PropTypes.object.isRequired,
};
const styles = theme => ({
root:{
margin: theme.spacing.unit * 4
},
aboutText:{
minWidth: 500,
maxWidth: 1000,
}
});
export default withStyles(styles)(About);<file_sep>import React from 'react';
import PropTypes from 'prop-types';
import {withStyles} from '@material-ui/core/styles';
import Snackbar from '@material-ui/core/Snackbar';
import SnackbarContent from '@material-ui/core/SnackbarContent';
import CloseIcon from '@material-ui/icons/Close';
import IconButton from '@material-ui/core/IconButton';
import green from '@material-ui/core/colors/green';
import amber from '@material-ui/core/colors/amber';
import NotificationContext from './NotificationContext';
import { getErrorMessage } from '../../utils/errorMessageHelper';
// show success/warning/error snackbar in right lower corner of viewport
class NotificationSnackbar extends React.Component {
handleChange = name => event => {
this.setState({
[name]: event.target.value,
});
};
// do not show Snackbar anymore
handleClose = (event, reason) => {
if(reason === 'clickaway') {
return;
}
this.context.handleNotificationChange(false,'','','');
};
render() {
const {classes} = this.props;
const { error, message, statusCode, action, variant } = this.context;
return (
<div>
<Snackbar
anchorOrigin={{
vertical: 'bottom',
horizontal: 'right',
}}
open={error}
autoHideDuration={8000}
resumeHideDuration={0}
disableWindowBlurListener={true}
onClose={this.handleClose}
transitionDuration={0}
>
<SnackbarContent
classes={{root: classes[variant]}}
aria-describedby="snackbar"
message={<span id={'snackbar'}>{getErrorMessage(message, statusCode, action)}</span>}
action={[
<IconButton
key="close"
aria-label="Close"
color="inherit"
className={classes.close}
onClick={this.handleClose}
>
<CloseIcon className={classes.icon} />
</IconButton>,
]}
/>
</Snackbar>
</div>
)
}
}
NotificationSnackbar.propTypes = {
classes: PropTypes.object.isRequired,
};
NotificationSnackbar.defaultProps = {
message: 'Es ist ein Fehler aufgetreten.',
};
NotificationSnackbar.contextType = NotificationContext;
const styles = theme => ({
root: {},
error: {
backgroundColor: theme.palette.error.dark,
},
success: {
backgroundColor: green[600],
},
warning: {
backgroundColor: amber[700],
},
icon: {
fontSize: 20,
},
iconVariant: {
opacity: 0.9,
marginRight: theme.spacing.unit,
},
});
export default withStyles(styles)(NotificationSnackbar);<file_sep>import React from 'react';
import PropTypes from 'prop-types';
import { withStyles } from '@material-ui/core/styles';
import Input from '@material-ui/core/Input';
import InputLabel from '@material-ui/core/InputLabel';
import MenuItem from '@material-ui/core/MenuItem';
import FormControl from '@material-ui/core/FormControl';
import ListItemText from '@material-ui/core/ListItemText';
import Select from '@material-ui/core/Select';
import Checkbox from '@material-ui/core/Checkbox';
const ITEM_HEIGHT = 48;
const ITEM_PADDING_TOP = 8;
const MenuProps = {
PaperProps: {
style: {
maxHeight: ITEM_HEIGHT * 4.5 + ITEM_PADDING_TOP,
width: 250,
},
},
};
class GenreSelection extends React.PureComponent {
state = {
selectedGenre: this.props.initialValues,
genres: this.props.genres,
};
// update state with new genres if prop changes
componentWillReceiveProps = nextProps => {
if(this.props.genres !== nextProps.genres) {
this.setState({genres: nextProps.genres});
}
};
handleChange = event => {
this.setState({ selectedGenre: event.target.value }, () => {
this.props.onInputChange(this.props.cardId, 'genres', this.state.selectedGenre); // update SearchCard state
});
};
render() {
const { classes, variant, disabled } = this.props;
const { genres, selectedGenre } = this.state;
return(
<div className={classes.root}>
<FormControl variant={variant} fullWidth={true} disabled={disabled}>
<InputLabel htmlFor="selectGenre">Genre(s)</InputLabel>
<Select
multiple
value={selectedGenre}
onChange={this.handleChange}
input={<Input id="selectGenre" />}
renderValue={selected => selected.join(', ')}
MenuProps={MenuProps}
>
{genres && Array.isArray(genres) && genres.map(name => (
<MenuItem key={name} value={name}>
<Checkbox
checked={selectedGenre.indexOf(name) > -1}
disableRipple={true}
color={"primary"}
/>
<ListItemText primary={name} />
</MenuItem>
))}
</Select>
</FormControl>
</div>
);
}
}
GenreSelection.propTypes = {
classes: PropTypes.object.isRequired,
genres: PropTypes.arrayOf(PropTypes.string),
cardId: PropTypes.string.isRequired,
variant: PropTypes.string.isRequired,
initialValues: PropTypes.array.isRequired,
disabled: PropTypes.bool.isRequired,
onInputChange: PropTypes.func.isRequired,
};
const styles = theme => ({
root: {
flexGrow: 1,
paddingTop: 5,
marginLeft: theme.spacing.unit,
marginRight: theme.spacing.unit,
position: 'relative',
},
});
export default withStyles(styles)(GenreSelection);<file_sep>import React from 'react';
import { withStyles } from '@material-ui/core/styles';
import theme from './theme/theme'
import { MuiThemeProvider } from '@material-ui/core/styles';
import {
BrowserRouter as Router,
Route,
Redirect,
Switch,
} from 'react-router-dom';
import NotificationContext from './components/notifications/NotificationContext';
import Header from './components/views/navigation/Header';
import Login from './components/views/login/Login';
import Browser from './components/views/browser/Browser';
import About from './components/views/About';
import Admin from './components/views/admin/Admin';
import MissingPage from './components/views/MissingPage';
import Notification from './components/notifications/NotificationSnackbar';
import Downloads from './components/views/Downloads';
// App component
class App extends React.Component {
state = {
loggedIn: false,
sessionID: 0,
isAdmin: false,
timeRange: {
minYear: '',
maxYear: '',
},
authors: [],
genres: ['poem', 'ballad', 'sonnet'],
notification: {
show: false,
statusCode: 0,
message: 'notification',
action: '',
variant: 'error',
}
};
// set state of App component
handleStateChange = (prop, value) => {
this.setState({
[prop]: value,
}, () => {
if(prop === 'sessionID' && this.state.loggedIn === true) {
this.requestSuggestions();
}
});
};
// open/close notification snackbar, display message (depending on optional statusCode and variant)
handleNotificationChange = (show, message, action, variant, statusCode, ) => {
this.handleStateChange('notification', {
show: show,
statusCode: statusCode? statusCode : 0,
message: message? message : '',
action: action,
variant: variant? variant : 'error',
});
};
requestSuggestions = () => {
// request initial data (authors, genres, time range, user status
this.requestAuthors();
this.requestLog();
};
// request authors list
requestAuthors = () => {
fetch("/backend/lib/functions.php", {
method: 'POST',
credentials: 'same-origin', // allow cookies -> session management
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({
authors: true,
id: this.state.sessionID,
})
}).then(response => {
if(response.ok) {
response.json().then(data => {
if(data && data.status === 'success' && data.authors) {
this.handleStateChange('authors', JSON.parse(data.authors));
} else {
// server error
this.handleNotificationChange(true, 'Autoren/Genres/Zeitspanne konnten nicht vom Server geladen werden.', 'initialLoad', 'error');
}
});
} else {
this.handleNotificationChange(true, 'Autoren/Genres/Zeitspanne konnten nicht vom Server geladen werden.', 'initialLoad', 'error');
}
}
).catch(error => {
this.handleNotificationChange(true, 'Autoren/Genres/Zeitspanne konnten nicht vom Server geladen werden.', 'initialLoad', 'error', 404);
}
);
};
// request genres + time range
requestLog = () => {
fetch("/backend/lib/functions.php", {
method: 'POST',
credentials: 'same-origin', // allow cookies -> session management
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({
log: true,
id: this.state.sessionID
})
}).then(response => {
if(response.ok) {
response.json().then(data => {
if(data && data.status === 'success' && data.log) {
let parsedLog = JSON.parse(data.log);
let parsedGenre = parsedLog.genre? parsedLog.genre.replace('[','').replace(']','').split(',') : [];
let parsedMinYear = parsedLog.minYear? parsedLog.minYear.slice(0,4) : '';
let parsedMaxYear = parsedLog.maxYear? parsedLog.maxYear.slice(0,4) : '';
this.handleStateChange('genres', parsedGenre);
this.handleStateChange('timeRange', {minYear: parsedMinYear, maxYear: parsedMaxYear});
} else {
this.handleNotificationChange(true, 'Autoren/Genres/Zeitspanne konnten nicht vom Server geladen werden.', 'initialLoad', 'error');
}
});
} else {
this.handleNotificationChange(true, 'Autoren/Genres/Zeitspanne konnten nicht vom Server geladen werden.', 'initialLoad', 'error');
}
}
).catch(error => {
this.handleNotificationChange(true, 'Autoren/Genres/Zeitspanne konnten nicht vom Server geladen werden.', 'initialLoad', 'error', 404);
}
);
};
// executed after component is inserted into the tree
componentDidMount = () => {
// request initial data (authors, genres, time range, user status
this.requestAuthors();
this.requestLog();
};
// logout user, request server to delete sessionID, display error if necessary
logout = () => {
fetch('/backend/lib/sessionManagement.php', {
method: 'POST',
credentials: 'same-origin', // allow cookies -> session management
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({
logout: true,
loginID: this.state.sessionID
})
})
.then(response => {
if(response.ok && response.json().status === 'success') {
this.handleNotificationChange(true, 'Logout erfolgreich', 'logout', 'success');
}
this.setState({
loggedIn: false,
isAdmin: false,
});
})
.catch(error => {
this.handleNotificationChange(true, error.message, 'logout', 'error', 404);
this.setState({
loggedIn: false,
isAdmin: false,
});
});
};
render() {
const { classes } = this.props;
const { loggedIn, isAdmin, authors, timeRange, notification, genres, sessionID } = this.state;
return (
<MuiThemeProvider theme={theme}>
<NotificationContext.Provider
value={{
error: notification.show,
message: notification.message,
statusCode: notification.statusCode,
action: notification.action,
variant: notification.variant,
handleNotificationChange: this.handleNotificationChange,
}}
>
<div className={classes.root}>
<Router>
<div>
<Header loggedIn={loggedIn} isAdmin={isAdmin} logout={this.logout}/>
<div className={classes.contentWrapper}>
<Switch>
<Route exact path='/' render={() => (
loggedIn? (<Browser sessionID={sessionID} handleAppChange={this.handleStateChange} authorsList={authors} minYear={timeRange.minYear} maxYear={timeRange.maxYear} genres={genres}/>) :
(<Redirect to='/login'/>)
)}/>
<Route path='/about' component={About}/>
<Route path='/downloads' render={() => (
loggedIn? (<Downloads sessionID={sessionID} handleAppChange={this.handleStateChange}/>) : (<Redirect to='/login'/>)
)}/>
<Route path='/admin' render={() => (
(loggedIn && isAdmin)?
(<Admin
requestNewAuthors={this.requestAuthors}
requestNewLog={this.requestLog}
sessionID={sessionID}
handleAppChange={this.handleStateChange}/>) :
(<Redirect to='/' />)
)}/>
<Route path='/login' render={() => (
loggedIn? (<Redirect to='/'/>) : (<Login handleAppStateChange={this.handleStateChange} />)
)}/>
<Route component={MissingPage}/>
</Switch>
</div>
<Notification />
</div>
</Router>
</div>
</NotificationContext.Provider>
</MuiThemeProvider>
);
}
}
const styles = {
root: {
height: '100%',
},
contentWrapper: {
height: '100%',
paddingTop: 0,
},
routerWrapper: {
height: '100%',
},
};
export default withStyles(styles)(App);
<file_sep>import React from 'react';
import PropTypes from 'prop-types';
import deburr from 'lodash/deburr';
import Downshift from 'downshift';
import keycode from 'keycode';
import { withStyles } from '@material-ui/core/styles';
import TextField from '@material-ui/core/TextField';
import Chip from '@material-ui/core/Chip';
import Paper from '@material-ui/core/Paper';
import MenuItem from '@material-ui/core/MenuItem';
// Quelle: https://material-ui.com/demos/autocomplete/ (Stand: Dezember 2018, mit eigenen Veränderungen)
// render input field for user
const InputField = inputProps => {
const { InputProps, classes, ref, ...other } = inputProps;
return (
<TextField
variant={inputProps.variant}
InputLabelProps={{disableAnimation: false,}}
InputProps={{
inputRef: ref,
classes: {
root: classes.inputRoot,
input: classes.inputInput,
},
...InputProps,
}}
{...other}
/>
);
};
// render author list to be displayed below input field
const Suggestion = ({ suggestion, index, itemProps, highlightedIndex, selectedItem }) => {
const isHighlighted = highlightedIndex === index;
const isSelected = (selectedItem || '').indexOf(suggestion) > -1;
return (
<MenuItem
{...itemProps}
key={suggestion}
selected={isHighlighted}
component="div"
style={{
fontWeight: isSelected ? 500 : 400,
}}
>
{suggestion}
</MenuItem>
);
};
Suggestion.propTypes = {
highlightedIndex: PropTypes.number,
index: PropTypes.number,
itemProps: PropTypes.object,
selectedItems: PropTypes.string,
suggestion: PropTypes.string.isRequired,
};
// render AuthorInput component
class AuthorInput extends React.PureComponent {
state = {
inputValue: '',
selectedItems: this.props.initialValues, // shape: ['Goethe, <NAME>', 'Rilke, <NAME>']
};
// handle pressing of keys (used to detect user deleting with backspace key)
handleKeyDown = event => {
const { inputValue, selectedItems } = this.state;
if (selectedItems.length && !inputValue.length && keycode(event) === 'backspace') {
this.setState({
selectedItems: selectedItems.slice(0, selectedItems.length - 1),
});
}
};
// extract suggestions from authorsList (max. 10 suggestions are being displayed)
getSuggestions = value => {
if(!value && value !== '') return ;
const inputValue = deburr(value.trim()).toLowerCase();
const inputLength = inputValue.length;
let count = 0;
return inputLength === 0 ? [] : this.props.authorsList.filter(author => {
const keep = (count < 10 && author.toLowerCase().includes(inputValue)
&& this.state.selectedItems.indexOf(author) === -1);
if (keep) {
count += 1;
}
return keep;
});
};
// set new state when input changes (not selected authors)
handleInputChange = event => {
this.setState({ inputValue: event.target.value });
};
// add new selected author to list that will be used for search
handleChange = item => {
let { selectedItems } = this.state;
// allow a max. of 10 authors per search card
if (selectedItems.length === 10) return;
if (selectedItems.indexOf(item) === -1) {
selectedItems = [...selectedItems, item];
}
this.setState({
inputValue: '',
selectedItems: selectedItems,
}, () => {
this.props.onInputChange(this.props.cardId, 'authors', this.state.selectedItems); // update SearchCard state
});
};
// delete selected authors from state => not search for them
handleDelete = item => () => {
this.setState(state => {
const selectedItem = [...state.selectedItems];
selectedItem.splice(selectedItem.indexOf(item), 1);
return { selectedItems: selectedItem };
}, () => {
this.props.onInputChange(this.props.cardId, 'authors', this.state.selectedItems); // update SearchCard state
});
};
render() {
const { classes, variant, disabled, autofocus } = this.props;
const { inputValue, selectedItems } = this.state;
return(
<div className={classes.root}>
<Downshift inputValue={inputValue} onChange={this.handleChange} selectedItem={selectedItems}>
{({getInputProps, getItemProps, isOpen, inputValue: inputValue2, selectedItem: selectedItem2,
highlightedIndex,}) => (
<div className={classes.container}>
{InputField({
fullWidth: true,
autoFocus: autofocus,
classes,
InputProps: getInputProps({
startAdornment: selectedItems.map(item => (
<Chip
key={item}
tabIndex={-1}
label={item}
className={classes.chip}
onDelete={this.handleDelete(item)}
/>
)),
onChange: this.handleInputChange,
onKeyDown: this.handleKeyDown,
value: inputValue,
placeholder: 'Bsp.: Rilke, <NAME>',
}),
label: 'Autor*in',
variant: variant,
disabled: disabled,
})}
{isOpen ? (
<Paper className={classes.paper} square>
{this.getSuggestions(inputValue2).map((suggestion, index) =>
Suggestion({
suggestion,
index,
itemProps: getItemProps({ item: suggestion }),
highlightedIndex,
selectedItem: selectedItem2,
}),
)}
</Paper>
) : null}
</div>
)}
</Downshift>
</div>
);
}
}
AuthorInput.propTypes = {
classes: PropTypes.object.isRequired,
cardId: PropTypes.string.isRequired,
variant: PropTypes.string,
initialValues: PropTypes.arrayOf(PropTypes.string).isRequired,
autofocus: PropTypes.bool.isRequired,
disabled: PropTypes.bool.isRequired,
onInputChange: PropTypes.func.isRequired,
authorsList: PropTypes.arrayOf(PropTypes.string),
};
const styles = theme => ({
root: {
flexGrow: 1,
paddingTop: 5,
},
container: {
marginLeft: theme.spacing.unit,
marginRight: theme.spacing.unit,
flexGrow: 1,
position: 'relative',
},
paper: {
position: 'absolute',
zIndex: 1,
marginTop: theme.spacing.unit,
left: 0,
right: 0,
},
chip: {
margin: `${theme.spacing.unit / 2}px ${theme.spacing.unit / 4}px`,
},
inputRoot: {
flexWrap: 'wrap',
},
inputInput: {
width: 'auto',
flexGrow: 1,
},
});
export default withStyles(styles)(AuthorInput);
<file_sep>import React from 'react';
import PropTypes from 'prop-types';
import { withStyles } from '@material-ui/core/styles';
import Typography from "@material-ui/core/Typography/Typography";
import Paper from "@material-ui/core/Paper/Paper";
import InfoButton from '@material-ui/icons/Info';
function InfoCard(props) {
const { classes, message } = props;
return(
<Paper className={classes.infoBox}>
<InfoButton color={"primary"} className={classes.infoIcon}/>
<Typography color={"primary"}>
{message}
</Typography>
</Paper>
)
}
InfoCard.propTypes = {
classes: PropTypes.object.isRequired,
message: PropTypes.string,
};
const styles = theme => ({
infoBox:{
minWidth: 400,
maxWidth: 800 + theme.spacing.unit*7,
padding: 20,
display: 'flex',
marginLeft: theme.spacing.unit,
marginBottom: theme.spacing.unit * 3,
},
infoIcon: {
marginRight: theme.spacing.unit,
},
});
export default withStyles(styles)(InfoCard);<file_sep>import React from 'react';
import PropTypes from 'prop-types';
import {withStyles} from '@material-ui/core/styles';
import Button from "@material-ui/core/Button";
import NotificationContext from '../../../notifications/NotificationContext';
import InfoCard from '../../../InfoCard';
// "Server verwalten" tab in Admin View
class ServerManagement extends React.Component {
state = {
loading: false,
};
handleChange = (prop, value) => {
this.setState({
[prop]: value,
});
};
// request server to empty its cache
requestEmptyCache = () => {
this.handleChange('loading', true);
fetch(' /backend/lib/admin.php', {
method: 'POST',
credentials: 'same-origin', // allow cookies -> session management
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({
cache: true,
})
})
.then(response => {
if (response.ok) {
response.json().then(data => {
if (data && data.status === 'success') {
// cache was emptied
this.context.handleNotificationChange(true, 'Der Server-Cache wurde geleert.', 'emptyCache', 'success');
} else {
this.context.handleNotificationChange(true, 'Der Server-Cache konnte nicht geleert werden.', 'emptyCache', 'error');
}
})
} else {
this.context.handleNotificationChange(true, 'Der Server-Cache konnte nicht geleert werden.', 'emptyCache', 'error');
}
this.handleChange('loading', false);
})
.catch(error => {
this.context.handleNotificationChange(true, 'Der Server-Cache konnte nicht geleert werden.', 'emptyCache', 'error');
this.handleChange('loading', false);
});
};
render() {
const {classes, requestStatus} = this.props;
const {loading} = this.state;
return (
<div>
<InfoCard message={'Hier können Sie den Cache der Suchaufrufe auf dem Server leeren.'}/>
<Button
color={'primary'}
variant={'contained'}
disabled={loading}
className={classes.cacheButton}
onClick={() => requestStatus(this.requestEmptyCache)}
>
Cache Leeren
</Button>
</div>
)
}
}
ServerManagement.propTypes = {
classes: PropTypes.object.isRequired,
requestStatus: PropTypes.func.isRequired,
};
ServerManagement.contextType = NotificationContext;
const styles = theme => ({
serverPaper: {
marginTop:'10px',
marginRight: '20px',
},
serverBox: {
padding: 5,
display: 'flex',
},
cacheButton: {
margin: theme.spacing.unit,
}
});
export default withStyles(styles)(ServerManagement);<file_sep>import React from 'react';
import PropTypes from 'prop-types';
import { withStyles } from '@material-ui/core/styles';
import TextField from '@material-ui/core/TextField';
import InputAdornment from '@material-ui/core/InputAdornment';
import IconButton from '@material-ui/core/IconButton';
import InfoIcon from '@material-ui/icons/Info';
import Typography from '@material-ui/core/Typography';
import Dialog from '@material-ui/core/Dialog';
class ContainsInput extends React.PureComponent {
state = {
keywords: this.props.initialValue,
dialogOpen: false,
syntaxError: false,
errorMessage: 'Fehler',
};
onInputChange = (event) => {
this.setState({keywords: event.target.value,}, () => {
this.props.onInputChange(this.props.cardId, 'keywords', this.state.keywords); // update SearchCard
});
};
onFocus = () => {
this.setState({syntaxError: false})
};
onOpenDialog = () => {
this.setState({dialogOpen: true})
};
onCloseDialog = () => {
this.setState({dialogOpen: false})
};
checkInput = () => {
let error = false;
let errorMessage = '';
//let string = this.state.keywords;
this.setState({
syntaxError: error,
errorMessage: errorMessage,
}, () => {
this.props.handleBrowserChange('keywordError', error);
});
};
render() {
const { classes, variant, disabled } = this.props;
const { keywords, syntaxError } = this.state;
return(
<div className={classes.root}>
<TextField
id="textContains"
label="Text enthält"
placeholder="Geben Sie Stichwörter ein"
multiline
fullWidth={true}
className={classes.textField}
variant={variant}
disabled={disabled}
error={syntaxError}
value={keywords}
onChange={this.onInputChange}
onFocus={this.onFocus}
onBlur={this.checkInput}
InputProps={{
endAdornment: (
<InputAdornment position="end">
<IconButton onClick={this.onOpenDialog}>
<InfoIcon />
</IconButton>
</InputAdornment>)}}
/>
{(this.state.syntaxError || this.state.timeFromError) &&
<Typography color={'error'} className={classes.errorMessage}>{this.state.errorMessage}</Typography>}
<Dialog
open={this.state.dialogOpen}
onClose={this.onCloseDialog}
aria-labelledby="dialogTitle"
className={classes.dialogWrapper}
>
<Typography variant={"h6"} color="primary" className={classes.listHeader}>Hinweise zum "Text enthält" Suchfeld</Typography>
<div className={classes.listWrapper}>
<ul>
<Typography color={"error"}>
<b>Achtung: Durch umfangreiche Eingaben wird die Suche auf dem Server sehr speicherintensiv und kann eventuell nicht ausgeführt werden.
Liefert eine Anfrage keine Ergebnisse, so kann dies auch an einer falschen Syntax in diesem Feld liegen.</b>
</Typography>
<li>
<Typography>Geben Sie <b>einzelne Wörter oder Phrasen</b> an.</Typography>
<Typography color={"textSecondary"}>Bsp.: Der Frühling ist schön</Typography>
<Typography color={"textSecondary"}>Ergebnis: Texte, die die exakte Phrase enthalten. Groß- und Kleinschreibung wird nicht beachtet.</Typography>
</li>
<li>
<Typography>Wenn Sie Phrasen suchen, die mit einem bestimmten Wort anfangen, kennzeichnen Sie dies mit einem <b>Stern</b> (*) am Ende der Phrase.</Typography>
<Typography color={"textSecondary"}>Bsp.: Blume*</Typography>
<Typography color={"textSecondary"}>Ergebnis: Texte mit dem Wort Blume und auch z.B. Blumenstrauß, aber nicht Ringelblume.</Typography>
</li>
<li>
<Typography>Verbinden Sie Phrasen durch ein <b>"and"</b>.</Typography>
<Typography color={"textSecondary"}>Bsp.: Frühling "and" Sonne</Typography>
<Typography color={"textSecondary"}>Ergebnis: Texte, die die Worte "Frühling" und "Sonne" gleichzeitig enthalten.</Typography>
</li>
<li>
<Typography>Verwenden Sie <b>"or"</b>, um nach alternativen Phrasen zu suchen. Sie können bis zu 3 Mal in einer Teil-Suche "or" verwenden.</Typography>
<Typography color={"textSecondary"}>Bsp.: Frühling "or" Sonne</Typography>
<Typography color={"textSecondary"}>Ergebnis: Texte, die mindestens eins der Worte "Frühling" und "Sonne" enthalten.</Typography>
<Typography color={"textSecondary"}>Bsp.: Frühling "or" Sonne "or" die Welt ist schön "or" die Blumen blühen</Typography>
<Typography color={"textSecondary"}>Ergebnis: Texte, die mindestens eine der 4 Wörter/Phrasen enthalten.</Typography>
<Typography color={"textSecondary"}>Bsp.: Frühling "or" Sonne "and" Liebe</Typography>
<Typography color={"textSecondary"}>Ergebnis: Texte, die mindestens eins der Worte "Frühling" oder "Sonne" und "Liebe" (die letzten beiden gleichzeitig, falls das erste nicht enthalten ist) enthalten.</Typography>
</li>
<li>
<Typography>Schließen Sie Phrasen mit <b>"not"</b> von der Suche aus. Achtung: "not" soll immer am Ende der Eingabe (ggf. nach "or" oder "and") stehen.</Typography>
<Typography color={"textSecondary"}>Bsp.: Frühling "or" Sonne "not" Liebe</Typography>
<Typography color={"textSecondary"}>Ergebnis: Texte, die mindestens eins der Wörter "Frühling" oder "Sonne" und gleichzeitig nicht das Wort "Liebe" enthält</Typography>
</li>
</ul>
</div>
</Dialog>
</div>
);
}
}
ContainsInput.propTypes = {
classes: PropTypes.object.isRequired,
cardId: PropTypes.string.isRequired,
variant: PropTypes.string.isRequired,
initialValue: PropTypes.string.isRequired,
disabled: PropTypes.bool.isRequired,
onInputChange: PropTypes.func.isRequired,
handleBrowserChange: PropTypes.func.isRequired,
};
const styles = theme => ({
root:{
flexGrow: 1,
paddingTop: 5,
marginLeft: theme.spacing.unit,
marginRight: theme.spacing.unit,
position: 'relative',
},
textField:{
//marginTop: theme.spacing.unit,
},
listWrapper:{
margin: theme.spacing.unit * 2,
marginRight: theme.spacing.unit * 4,
marginTop: -3 * theme.spacing.unit,
minWidth: 400,
},
dialogHeader:{
marginLeft: theme.spacing.unit * 4,
marginTop: theme.spacing.unit,
},
listHeader:{
marginLeft: theme.spacing.unit * 4,
marginTop: theme.spacing.unit * 4,
marginBottom: theme.spacing.unit * 2,
},
dialogWrapper:{
minWidth: 600,
},
errorMessage:{
fontSize: '0.75rem',
}
});
export default withStyles(styles)(ContainsInput);<file_sep>import React from 'react';
import PropTypes from 'prop-types';
import {withStyles} from '@material-ui/core/styles';
import { Link } from "react-router-dom";
import Drawer from '@material-ui/core/Drawer';
import List from '@material-ui/core/List';
import ListItem from '@material-ui/core/ListItem';
import ListItemText from '@material-ui/core/ListItemText';
import Typography from '@material-ui/core/Typography';
class AdminNav extends React.Component {
state = {
selectedItem: -1,
};
handleChange = (prop, value) => {
this.setState({
[prop]: value,
});
};
handleSelectChange = (item) => {
this.handleChange('selectedItem', item);
};
render() {
const {classes} = this.props;
const {selectedItem} = this.state;
return (
<div className={classes.root}>
<Drawer
className={classes.drawer}
variant="permanent"
classes={{
paper: classes.drawerPaper,
}}
anchor="left"
>
<List className={classes.navElements}>
<ListItem button component={Link} to='/admin' color='primary' selected={selectedItem === 0}>
<ListItemText primary={<Typography color={'primary'} variant={'h6'}>Texte verwalten</Typography>}/>
</ListItem>
<ListItem button component={Link} to='/admin/users' color='primary' selected={selectedItem === 1}>
<ListItemText primary={<Typography color={'primary'} variant={'h6'}>User verwalten</Typography>} />
</ListItem>
<ListItem button component={Link} to='/admin/server' color='primary' selected={selectedItem === 2}>
<ListItemText primary={<Typography color={'primary'} variant={'h6'}>Server verwalten</Typography>}/>
</ListItem>
</List>
</Drawer>
</div>
)
}
}
AdminNav.propTypes = {
classes: PropTypes.object.isRequired,
};
const styles = ({
root: {
width: 'auto',
height: '100%',
//zIndex: 1,
},
navElements:{
paddingTop: 100,
height: 150,
},
navElement:{
//display:'block',
},
drawer:{
width: 210,
flexShrink: 0,
},
drawerPaper:{
width: 210,
backgroundColor: '#e8e8e8',
},
});
export default withStyles(styles)(AdminNav);<file_sep>import React from 'react';
import PropTypes from 'prop-types';
import {withStyles} from '@material-ui/core/styles';
import Button from "@material-ui/core/Button";
import TextField from '@material-ui/core/TextField';
import Paper from "@material-ui/core/Paper/Paper";
import Table from '@material-ui/core/Table';
import TableBody from '@material-ui/core/TableBody';
import TableCell from '@material-ui/core/TableCell';
import TableHead from '@material-ui/core/TableHead';
import TableRow from '@material-ui/core/TableRow';
import NotificationContext from '../../../notifications/NotificationContext';
import InfoCard from '../../../InfoCard';
import Typography from "@material-ui/core/Typography/Typography";
import IconButton from '@material-ui/core/IconButton';
import DeleteIcon from '@material-ui/icons/Delete';
import FormControlLabel from '@material-ui/core/FormControlLabel';
import Checkbox from '@material-ui/core/Checkbox';
class UserManagement extends React.Component {
componentDidMount = () => {
// load user list
this.props.requestStatus(this.requestUsers);
};
state = {
loading: false,
users: [{user: 'exampleUser', isAdmin: true}],
isOpen: false,
newUser: {
name: '',
pw1: '',
pw2: '',
isAdmin: false,
}
};
handleChange = (prop, value) => {
this.setState({
[prop]: value,
});
};
handleUserChange = (event) => {
let newUser = this.state.newUser;
let name = event.target.name;
newUser[name] = name === 'isAdmin'? event.target.checked : event.target.value;
this.handleChange('newUser', newUser);
};
// check how many admins are in the users list
getAdminNumber = users => users.filter(user => user.isAdmin).length;
// check username min length of 3 characters and max length of 30 characters
checkUsernameLength = username => username.length > 2 && username.length < 31;
// compare passwords (before sending)
comparePasswords = (pw1, pw2) => {
return pw1 === pw2;
};
// Is password long enough?
checkPasswordLength = password => password.length < 41 && password.length > 3;
// request users list from server
requestUsers = () => {
this.handleChange('loading', true);
fetch('/backend/lib/admin.php', {
method: 'POST',
credentials: 'same-origin', // allow cookies -> session management
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({
users: true,
}),
})
.then(response => {
if (response.ok) {
response.json().then(data => {
if (data && data.status === 'success') {
// received users list from server
this.handleChange('users', JSON.parse(data.users));
} else {
this.context.handleNotificationChange(true, 'Die Nutzerliste konnte nicht geladen werden.', 'loadUsers', 'error');
}
})
} else {
this.context.handleNotificationChange(true, 'Die Nutzerliste konnte nicht geladen werden.', 'loadUsers', 'error');
}
this.handleChange('loading', false);
})
.catch(error => {
this.context.handleNotificationChange(true, 'Die Nutzerliste konnte nicht geladen werden.', 'loadUsers', 'error');
this.handleChange('loading', false);
});
};
// create new user on server
requestNewUser = () => {
// check inputs before sending request
if(!this.checkUsernameLength(this.state.newUser.name)
|| !this.checkPasswordLength(this.state.newUser.pw1) || !this.checkPasswordLength(this.state.newUser.pw2)
|| !this.comparePasswords(this.state.newUser.pw1, this.state.newUser.pw2)
) {
this.context.handleNotificationChange(true, 'Bitte geben Sie gültige Nutzerdaten ein.', 'newUser', 'error');
return;
}
this.handleChange('loading', true);
fetch('/backend/lib/admin.php', {
method: 'POST',
credentials: 'same-origin', // allow cookies -> session management
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({
username: this.state.newUser.name,
password: <PASSWORD>,
isadmin: this.state.newUser.isAdmin === false ? 0 : 1,
}),
})
.then(response => {
if (response.ok) {
response.json().then(data => {
if (data && data.status === 'success') {
// created new user
this.props.requestStatus(this.requestUsers);
this.context.handleNotificationChange(true, 'Benutzer*in erfolgreich angelegt.', 'createUser', 'success');
} else {
this.context.handleNotificationChange(true, 'Benutzer*in konnte nicht angelegt werden.', 'createUser', 'error');
}
})
} else {
this.context.handleNotificationChange(true, 'Benutzer*in konnte nicht angelegt werden.', 'createUser', 'error');
}
this.handleChange('loading', false);
})
.catch(error => {
this.context.handleNotificationChange(true, 'Benutzer*in konnte nicht angelegt werden.', 'createUser', 'error');
this.handleChange('loading', false);
});
};
// delete user from server
requestDelete = username => {
if(!username) return;
if(this.state.users.filter(user => user.user === username)[0].isAdmin && this.getAdminNumber(this.state.users) === 1) {
this.context.handleNotificationChange(true, 'Legen Sie einen neuen Admin-Account an bevor Sie den einzigen löschen.', 'deleteUser', 'error');
return;
}
fetch('/backend/lib/admin.php', {
method: 'POST',
credentials: 'same-origin', // allow cookies -> session management
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({
delete: username,
})
})
.then(response => {
if (response.ok) {
response.json().then(data => {
if (data.status === 'success') {
this.props.requestStatus(this.requestUsers);
this.context.handleNotificationChange(true, 'Nutzer*in erfolgreich gelöscht.', 'deleteUser', 'success')
} else {
this.context.handleNotificationChange(true, 'Nutzer*in konnte nicht gelöscht werden.', 'deleteUser', 'error')
}
})
} else {
this.context.handleNotificationChange(true, 'Nutzer*in konnte nicht gelöscht werden.', 'deleteUser', 'error')
}
})
.catch(error => {
this.context.handleNotificationChange(true, 'Nutzer*in konnte nicht gelöscht werden.', 'deleteUser', 'error')
}
);
};
render() {
const { classes, requestStatus } = this.props;
const { loading, newUser, users } = this.state;
return (
<div>
<InfoCard message={'Hier können Sie Nutzer*innen hinzufügen und entfernen.'}/>
<div className={classes.marginLeftContainer}>
<Typography variant={'h6'} color={'primary'}>Registrierte Nutzer*innen</Typography>
<Paper className={classes.tableWrapper}>
<Table>
<TableHead>
<TableRow>
<TableCell scope={'column'}>Nr.</TableCell>
<TableCell scope={'column'}>Username</TableCell>
<TableCell scope={'column'}>Admin</TableCell>
<TableCell scope={'column'}>Aktion</TableCell>
</TableRow>
</TableHead>
<TableBody>
{users.map((user, index) => (
<TableRow key={user.user}>
<TableCell scope={'row'}>{index + 1}</TableCell>
<TableCell>{user.user}</TableCell>
<TableCell>{user.isadmin? 'Ja' : 'Nein'}</TableCell>
<TableCell className={classes.flexContainer}>
<IconButton onClick={() => requestStatus(() => this.requestDelete(user.user))}>
<DeleteIcon color={'secondary'}/>
</IconButton>
</TableCell>
</TableRow>
))}
</TableBody>
</Table>
</Paper>
</div>
<br/>
<div className={classes.marginLeftContainer}>
<Typography variant={'h6'} color={'primary'}>Nutzer*in hinzufügen</Typography>
<Paper className={classes.loginContainer}>
<div className={classes.formContainer}>
<Typography color={'primary'}>Bitte geben Sie Nutzerdaten ein:</Typography>
<TextField
disabled={loading}
autoFocus={true}
className={classes.textField}
label={'Benutzername'}
type={'text'}
value={newUser.name}
error={!loading && newUser.name !== '' && !this.checkUsernameLength(newUser.name)}
name={'name'}
onChange={this.handleUserChange}
/>
{!loading && newUser.name !== '' && !this.checkUsernameLength(newUser.name) && <Typography color={'error'} className={classes.errorMessage}>
Geben Sie mindestens 3 und höchstens 30 Zeichen ein.
</Typography>}
<TextField
className={classes.textField}
disabled={loading}
label={'Passwort'}
type={'password'}
name={'pw1'}
value={newUser.pw1}
error={!loading && newUser.pw1 !== '' && !this.checkPasswordLength(newUser.pw1)}
onChange={this.handleUserChange}
/>
{!loading && newUser.pw1 !== '' && !this.checkPasswordLength(newUser.pw1) && <Typography color={'error'} className={classes.errorMessage}>
Das Passwort muss mindestens 4 und höchstens 40 Zeichen lang sein.
</Typography>}
<TextField
className={classes.textField}
disabled={loading}
label={'Passwort wiederholen'}
type={'password'}
name={'pw2'}
value={newUser.pw2}
error={!loading && newUser.pw1 !== '' && !this.comparePasswords(newUser.pw1, newUser.pw2)}
onChange={this.handleUserChange}
/>
{!loading && !this.comparePasswords(newUser.pw1, newUser.pw2) && <Typography color={'error'} className={classes.errorMessage}>
Die Passwörter stimmen nicht überein.
</Typography>}
<FormControlLabel
control={
<Checkbox
checked={newUser.isAdmin}
onChange={this.handleUserChange}
name={'isAdmin'}
/>
}
label={'Admin'}/>
<div className={classes.flexContainer}>
<Button
disabled={loading}
size="small"
color="primary"
variant={"contained"}
className={classes.button}
onClick={() => requestStatus(this.requestNewUser)}
>
Hinzufügen
</Button>
</div>
</div>
</Paper>
</div>
<br/>
</div>
);
}
}
UserManagement.propTypes = {
classes: PropTypes.object.isRequired,
requestStatus: PropTypes.func.isRequired,
};
UserManagement.contextType = NotificationContext;
const styles = theme => ({
root: {
marginTop: theme.spacing.unit * 3,
overflowX: 'auto',
marginLeft: theme.spacing.unit,
marginRight: '20px',
width: 896,
},
marginLeftContainer: {
marginLeft: theme.spacing.unit,
},
table: {
minWidth: 400,
maxWidth: 800,
marginLeft: theme.spacing.unit,
},
newUser:{
padding: '20px',
marginTop: '20px'
},
nutzerpaper: {
marginTop:'10px',
marginRight: '20px',
},
nutzerBox: {
padding: 5,
display: 'flex',
},
tableWrapper: {
marginTop: theme.spacing.unit * 3,
overflowX: 'auto',
marginRight: '20px',
width: 896,
},
loginContainer:{
width: 400,
padding: theme.spacing.unit * 6,
marginTop: theme.spacing.unit * 2
},
formContainer:{
display: 'flex',
flexDirection: 'column',
},
textField: {
marginTop: theme.spacing.unit * 2,
},
button:{
marginTop: theme.spacing.unit * 5,
marginRight: theme.spacing.unit * 2,
width: 110,
padding: theme.spacing.unit,
},
flexContainer:{
display: 'flex',
alignItems: 'flex-end',
},
loginErrorMessage:{
marginLeft: -theme.spacing.unit,
marginTop: theme.spacing.unit * 3,
width: theme.spacing.unit * 52,
},
});
export default withStyles(styles)(UserManagement);
<file_sep>import React from 'react';
import PropTypes from 'prop-types';
import {withStyles} from '@material-ui/core/styles';
import Paper from "@material-ui/core/Paper/Paper";
import Typography from '@material-ui/core/Typography';
import Link from '@material-ui/core/Link';
import green from '@material-ui/core/colors/green';
import NotificationContext from '../../../notifications/NotificationContext';
// display search results + provide download link
class Results extends React.Component {
state = {};
shouldComponentUpdate = (nextProps) => {
// prevent links from updating after search is complete
return !(nextProps.formats !== this.props.formats
&& nextProps.searchID === this.props.searchID);
};
// see if user is still logged in
requestStatus = func => {
// check if session is still valid otherwise logout user
fetch("/backend/lib/sessionManagement.php", {
method: 'POST',
credentials: 'same-origin', // allow cookies -> session management
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({
loginStatus: true,
loginID: this.props.sessionID
})
}).then(response => {
if(response.ok) {
response.json().then(data => {
if(!data || data.status === 'error') {
this.context.handleNotificationChange(true, 'Ihre Sitzung ist abgelaufen.', 'sessionCheck', 'error');
this.props.handleAppChange('loggedIn', false);
this.props.handleAppChange('isAdmin', false);
} else {
func();
}
});
} else {
this.context.handleNotificationChange(true, 'Ihre Sitzung ist abgelaufen.', 'sessionCheck', 'error');
this.logout();
}
}
).catch(error => {
this.context.handleNotificationChange(true, 'Ihre Sitzung ist abgelaufen.', 'sessionCheck', 'error', 404);
this.logout();
}
);
};
downloadResults = (format,getAll) => {
if(getAll) {
let a = document.createElement('a');
a.href = ('/backend/archive/') + (format==='json'? 'corpusJson.zip' : 'corpusTxt.zip');
a.download = format==='json'? 'corpusJson' : 'corpusTxt';
a.click();
} else {
// request data + handle response for each selected format separately
fetch("/backend/lib/functions.php", {
method: 'POST',
credentials: 'same-origin', // allow cookies -> session management
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({
download: this.props.filenames,
format: format,
}),
})
.then(response => {
if(response.ok) {
response.json().then(data => {
if(data && data.status === 'success') {
// create invisible anchor and click it
let a = document.createElement('a');
const filename = data.filename.substr(0, data.filename.length-4) + (format === 'json'? 'Json' : 'Txt');
a.href = '/backend/database/_cache/' + data.filename;
a.download = filename;
a.click();
} else {
this.context.handleNotificationChange(true, 'Die Ergebnis-Dateien konnten nicht vom Server geladen werden.', 'download', 'error');
}
})
} else {
this.context.handleNotificationChange(true, 'Die Ergebnis-Dateien konnten nicht vom Server geladen werden.', 'download', 'error');
}
})
.catch((error) => {
this.context.handleNotificationChange(true, 'Die Ergebnis-Dateien konnten nicht vom Server geladen werden.', 'download', 'error');
});
}
};
render() {
const {classes, number, formats, getAll} = this.props;
return (
<div className={classes.root}>
<Paper className={classes.resultContainer} elevation={0} >
<Typography>
{formats.includes('json') &&
<Link
classes={{root: {cursor: 'pointer'}}}
className={classes.resultLink}
onClick={() => this.requestStatus(() => this.downloadResults('json', getAll))}
>
Suchergebnisse im JSON-Format herunterladen {!getAll && '(' + number + ' Treffer)'}
</Link>}
{formats.includes('txt') &&
<Link
classes={{root: {cursor: 'pointer'}}}
className={classes.resultLink}
onClick={() => this.requestStatus(() => this.downloadResults('txt', getAll))}
>
Suchergebnisse im TXT-Format herunterladen {!getAll && '(' + number + ' Treffer)'}
</Link>}
</Typography>
</Paper>
</div>
)
}
}
Results.propTypes = {
classes: PropTypes.object.isRequired,
filenames: PropTypes.string,
number: PropTypes.number.isRequired,
formats: PropTypes.arrayOf(PropTypes.string),
searchID: PropTypes.any.isRequired,
handleAppChange: PropTypes.func.isRequired,
sessionID: PropTypes.any.isRequired,
getAll: PropTypes.bool.isRequired,
};
Results.contextType = NotificationContext;
const styles = theme => ({
root: {
margin: theme.spacing.unit,
},
resultContainer:{
padding: theme.spacing.unit,
backgroundColor: green[600],
},
resultLink:{
color: 'white',
display: 'block',
cursor: 'pointer',
}
});
export default withStyles(styles)(Results); | e2270f34ecd48e9b6c59bf8348d6ceb689b5e1f6 | [
"JavaScript"
] | 19 | JavaScript | btepe/literature-browser | a1b4c63591bee45e26e5e848db49bb6d35a7baa8 | 7c2b82f29ad62a39726d087c19808d09b55f110e | |
refs/heads/master | <file_sep>var sdoc = sdoc + 1;
console.log("TC.JS +")
/*--------------------------------------*/
var TC1;
var TC2;
var TC3;
var TC4;
var TC5;<file_sep>var sdoc = sdoc + 1;
console.log("Logout.JS +")
/*--------------------------------------*/
function onLogout(){
$(".LoginPanel").show(2000);
$(".MainPanel").hide(2000);
$(".OpenPanel").hide(2000);
username = 0;
passkey = 0;
pin = 0;
dividepage = 0;
}<file_sep>var sdoc = sdoc + 1;
console.log("Account Types.JS +")
/*--------------------------------------*/
/*---------------------------*/
/*--Checking-Types-----------*/
/*---------------------------*/
var checkingtype1 = "Personal Checking";
var checkingtype2 = "Premium Checking";
/*---------------------------*/
/*--Savings-Types------------*/
/*---------------------------*/
var savingstype1 = "Personal Savings";
var savingstype2 = "Premium Savings";
<file_sep>var sdoc = sdoc + 1;
console.log("An Account JS Doc +")
/*--------------------------------------*/
var SystemAccount3 = "Account 2"
var SystemAccount3Number = "97232-79123-65212-89342";
var SystemAccount3Type = checkingtype1;
var endingn3 = SystemAccount3Number.substring(18,23);<file_sep>var sdoc = sdoc + 1;
console.log("Pagedivide.JS +")
/*--------------------------------------*/
function openSystem(){
$(".LoginPanel").hide(2000);
$(".MainPanel").hide(2000);
$(".OpenPanel").show(2000);
if (dividepage == 0){
window.onload = dividePage0();
}else if(dividepage == 1){
window.onload = dividePage1();
}else if(dividepage == 2){
window.onload = dividePage2();
}else if(dividepage == 3){
window.onload = dividePage3();
}else if(dividepage == 4){
window.onload = dividePage4();
}else if(dividepage == 5){
window.onload = dividePage5()
}
}
function dividePage0(){
$("#OP1").hide(0);
$("#OP2").hide(0);
$("#OP3").hide(0);
$("#OP4").hide(0);
$("#OP5").hide(0);
}
function dividePage1(){
$("#OP1").hide(0);
$("#OP2").hide(0);
$("#OP3").show(0);
$("#OP4").hide(0);
$("#OP5").hide(0);
}
function dividePage2(){
$("#OP1").hide(0);
$("#OP2").show(0);
$("#OP3").hide(0);
$("#OP4").show(0);
$("#OP5").hide(0);
}
function dividePage3(){
$("#OP1").hide(0);
$("#OP2").show(0);
$("#OP3").show(0);
$("#OP4").show(0);
$("#OP5").hide(0);
}
function dividePage4(){
$("#OP1").show(0);
$("#OP2").show(0);
$("#OP3").hide(0);
$("#OP4").show(0);
$("#OP5").show(0);
}
function dividePage5(){
$("#OP1").show(0);
$("#OP2").show(0);
$("#OP3").show(0);
$("#OP4").show(0);
$("#OP5").show(0);
}<file_sep>var sdoc = sdoc + 1;
console.log("An Account JS Doc +")
/*--------------------------------------*/
var SystemAccount1 = "Account 1"
var SystemAccount1Number = "23423-35345-24624-93857";
var SystemAccount1Type = checkingtype1;
var endingn1 = SystemAccount1Number.substring(18,23);
var Account1Balance = 330;<file_sep>var sdoc = sdoc + 1;
console.log("Active Account.JS +")
/*--------------------------------------*/
var ActiveAccountBalance;
var ActiveAccountB;<file_sep>var sdoc = sdoc + 1;
console.log("A User JS Doc +")
/*--------------------------------------*/
/*--User-Information--*/
var User1SA = ["guest","guest","1000"];
var User1SB = ["Demo","User","Guest","5/1/93"];
var User1SC = ["DGM-32451-GAZ"];
/*---Linked-Account---*/
/*-Max-Account-Link:6-*/
var User1LinkedAccount1 = checkingtype1;
var User1LinkedAccount1Key = endingn1;
var User1LinkedAccount2 = savingstype1;
var User1LinkedAccount2Key = endingn2;
var User1LinkedAccount3;
var User1LinkedAccount3Key;
var User1LinkedAccount4;
var User1LinkedAccount4Key;
var User1LinkedAccount5;
var User1LinkedAccount5Key;
<file_sep>var sdoc = 1;
console.log("Inital.JS +")
/*--------------------------------------*/
function onSystemboot(){
$(".LoginPanel").show(0);
$(".MainPanel").hide(0);
$(".OpenPanel").hide(0);
console.log(sdoc+""+" JS Doc's Linked");
}
function openSystem(){
$(".LoginPanel").hide(2000);
$(".MainPanel").hide(2000);
$(".OpenPanel").show(2000);
if (dividepage == 0){
window.onload = dividePage0();
}else if(dividepage == 1){
window.onload = dividePage1();
}else if(dividepage == 2){
window.onload = dividePage2();
}else if(dividepage == 3){
window.onload = dividePage3();
}else if(dividepage == 4){
window.onload = dividePage4();
}else if(dividepage == 5){
window.onload = dividePage5();
}
}
<file_sep>var sdoc = sdoc + 1;
console.log("An Account JS Doc +")
/*--------------------------------------*/
var SystemAccount2 = "Account 2"
var SystemAccount2Number = "23423-35345-24624-23007";
var SystemAccount2Type = checkingtype1;
var endingn2 = SystemAccount2Number.substring(18,23);<file_sep>var sdoc = sdoc + 1;
console.log("The Active User JS Doc +")
/*--------------------------------------*/
var LinkedAccount1;
var LinkedAccount1Key;
var LinkedAccount2;
var LinkedAccount2Key;
var LinkedAccount3;
var LinkedAccount3Key;
var LinkedAccount4;
var LinkedAccount4Key;
var LinkedAccount5;
var LinkedAccount5Key;
var dividepage = 0;
/*-------*/
function activeUser(){
if (User1SA[0] == username && User1SA[1] == passkey && login == true && dividepage == 0){
/*------------*/
LinkedAccount1 = User1LinkedAccount1;
LinkedAccount1Key = User1LinkedAccount1Key;
if (LinkedAccount1 != null|| LinkedAccount1 != undefined){
dividepage = dividepage + 1;
}
/*------------*/
LinkedAccount2 = User1LinkedAccount2;
LinkedAccount2Key = User1LinkedAccount2Key;
if (LinkedAccount2 != null|| LinkedAccount2 != undefined){
dividepage = dividepage + 1;
}
/*------------*/
LinkedAccount3 = User1LinkedAccount3;
LinkedAccount3Key = User1LinkedAccount3Key;
if (LinkedAccount3 != null|| LinkedAccount3 != undefined){
dividepage = dividepage + 1;
}
/*------------*/
LinkedAccount4 = User1LinkedAccount4;
LinkedAccount4Key = User1LinkedAccount4Key;
if (LinkedAccount4 != null|| LinkedAccount4 != undefined){
dividepage = dividepage + 1;
}
/*------------*/
LinkedAccount5 = User1LinkedAccount5;
LinkedAccount5Key = User1LinkedAccount5Key;
if (LinkedAccount5 != null|| LinkedAccount5 != undefined){
dividepage = dividepage + 1;
}
}else if (User2SA[0] == username && User2SA[1] == passkey && login == true && dividepage == 0){
/*------------*/
LinkedAccount1 = User2LinkedAccount1;
LinkedAccount1Key = User2LinkedAccount1Key;
if (LinkedAccount1 != null|| LinkedAccount1 != undefined){
dividepage = dividepage + 1;
}
/*------------*/
LinkedAccount2 = User2LinkedAccount2;
LinkedAccount2Key = User2LinkedAccount2Key;
if (LinkedAccount2 != null|| LinkedAccount2 != undefined){
dividepage = dividepage + 1;
}
/*------------*/
LinkedAccount3 = User2LinkedAccount3;
LinkedAccount3Key = User2LinkedAccount3Key;
if (LinkedAccount3 != null|| LinkedAccount3 != undefined){
dividepage = dividepage + 1;
}
/*------------*/
LinkedAccount4 = User2LinkedAccount4;
LinkedAccount4Key = User2LinkedAccount4Key;
if (LinkedAccount4 != null|| LinkedAccount4 != undefined){
dividepage = dividepage + 1;
}
/*------------*/
LinkedAccount5 = User2LinkedAccount5;
LinkedAccount5Key = User2LinkedAccount5Key;
if (LinkedAccount5 != null|| LinkedAccount5 != undefined){
dividepage = dividepage + 1;
}
}
window.onload = showAccount();
window.onload = openSystem();
}
function showAccount(){
if (login == true){
if(dividepage == 1){
$("#A3Info").text(LinkedAccount1);
$("#B3Info").text("Ending In:"+" "+LinkedAccount1Key);
}else if(dividepage == 2){
$("#A2Info").text(LinkedAccount1);
$("#B2Info").text("Ending In:"+" "+LinkedAccount1Key);
$("#A4Info").text(LinkedAccount2);
$("#B4Info").text("Ending In:"+" "+LinkedAccount2Key);
}else if(dividepage == 3){
$("#A2Info").text(LinkedAccount1);
$("#B2Info").text("Ending In:"+" "+LinkedAccount1Key);
$("#A3Info").text(LinkedAccount2);
$("#B3Info").text("Ending In:"+" "+LinkedAccount2Key);
$("#A4Info").text(LinkedAccount3);
$("#B4Info").text("Ending In:"+" "+LinkedAccount3Key);
}else if(dividepage == 4){
$("#A1Info").text(LinkedAccount1);
$("#B1Info").text("Ending In:"+" "+LinkedAccount1Key);
$("#A2Info").text(LinkedAccount2);
$("#B2Info").text("Ending In:"+" "+LinkedAccount2Key);
$("#A4Info").text(LinkedAccount4);
$("#B4Info").text("Ending In:"+" "+LinkedAccount5Key);
$("#A5Info").text(LinkedAccount5);
$("#B5Info").text("Ending In:"+" "+LinkedAccount5Key);
}else if(dividepage == 5){
$("#A1Info").text(LinkedAccount1);
$("#B1Info").text("Ending In:"+" "+LinkedAccount1Key);
$("#A2Info").text(LinkedAccount2);
$("#B2Info").text("Ending In:"+" "+LinkedAccount2Key);
$("#A3Info").text(LinkedAccount3);
$("#B3Info").text("Ending In:"+" "+LinkedAccount3Key);
$("#A4Info").text(LinkedAccount4);
$("#B4Info").text("Ending In:"+" "+LinkedAccount4Key);
$("#A5Info").text(LinkedAccount5);
$("#B5Info").text("Ending In:"+" "+LinkedAccount5Key);
}
}
}<file_sep># ATMOS
A small financial management app. Still in beta. (For now!)
| ae3a80eba3b409e412df0e3b6c44e513ae4f6c35 | [
"JavaScript",
"Markdown"
] | 12 | JavaScript | VOcchiogrosso/ATMOS | 967a4896d127b66c42314b7c58f3c19ec6f630a8 | e06aeb0ab5013a95500dd625b1bd9b77f41023d1 | |
refs/heads/master | <repo_name>qwerhacks/QWERHacks2022<file_sep>/src/components/Resources.js
import React from "react";
function Resources(props) {
return (
<div>
<img src={require("../img/mlh-trust-badge-2021-white.png")} alt="mlh badge" class="mlh" width="120px" />
<h1>RESOURCES</h1>
<div class="qanda">
<div class="question">
<a class="code-of-conduct" href="https://qwer-hacks-2021.devpost.com/project-gallery" target="_blank">project gallery!!!!</a>
</div>
<div class="answer">
<a class="code-of-conduct" href="https://qwer-hacks-2021.devpost.com/project-gallery" target="_blank">check out all of your project submissions (and winners!!!) from qwer hacks 2021</a>
</div>
</div>
{/* mlh code of conduct */}
<div class="qanda">
<div class="question">
<a class="code-of-conduct" href="https://static.mlh.io/docs/mlh-code-of-conduct.pdf" target="_blank">mlh code of conduct</a>
</div>
<div class="answer">
<a class="code-of-conduct" href="https://static.mlh.io/docs/mlh-code-of-conduct.pdf" target="_blank">please read through this before participating in our event!</a>
</div>
</div>
{/* how to safely attend qwerhacks */}
<div class="qanda">
<div class="question">
<a class="code-of-conduct" href="https://drive.google.com/file/d/15draOrpYUXQrvzcShnqW3ziShfaRQSP-/view?usp=sharing" target="_blank">how to safely attend our hackathon</a>
</div>
<div class="answer">
<a class="code-of-conduct" href="https://drive.google.com/file/d/15draOrpYUXQrvzcShnqW3ziShfaRQSP-/view?usp=sharing" target="_blank">
we affirm our commitment to making participation in this hackathon discreet, and we will work with participants every
step of the way to ensure their comfort and safety. in the following guide, we’re going to be offering our own tips,
tricks, and advice as to how you can attend this hackathon safely!
</a>
</div>
</div>
{/* how to attend a virtual hackathon */}
<div class="qanda">
<div class="question">
<a class="code-of-conduct" href="https://drive.google.com/file/d/1QV9o3asHa10RkXd8lsvC3fkjKIG7kq-z/view?usp=sharing" target="_blank">how to attend a virtual hackathon</a>
</div>
<div class="answer">
<a class="code-of-conduct" href="https://drive.google.com/file/d/1QV9o3asHa10RkXd8lsvC3fkjKIG7kq-z/view?usp=sharing" target="_blank">
Are you scared of attending your first hackathon? Are you a hackathon veteran who’s never attended a virtual one before? Then this guide is for you!
</a>
</div>
</div>
{/* judging criteria */}
{/* to survive on this shore */}
<div class="qanda">
<div class="question">
<a class="code-of-conduct" href="https://www.tosurviveonthisshore.com/" target="_blank">to survive on this shore</a>
</div>
<div class="answer">
<a class="code-of-conduct" href="https://www.tosurviveonthisshore.com/" target="_blank">
photographs and interviews with transgender and gender nonconforming older adults: a project by <NAME> and <NAME>
</a>
</div>
</div>
{/* creating a trans inclusive workspace */}
<div class="qanda">
<div class="question">
<a class="code-of-conduct" href="https://hbr.org/2020/03/creating-a-trans-inclusive-workplace" target="_blank">creating a trans inclusive workspace</a>
</div>
<div class="answer">
<a class="code-of-conduct" href="https://hbr.org/2020/03/creating-a-trans-inclusive-workplace" target="_blank">
only when people feel totally authentic and connected with their organizations can they achieve their full potential at work!
</a>
</div>
</div>
{/* te reo māori speech recognition: a story of community, trust, and sovereignty */}
<div class="qanda">
<div class="question">
<a class="code-of-conduct" href="https://www.youtube.com/watch?v=sGQy0r_icWc" target="_blank">te reo māori speech recognition: a story of community, trust, and sovereignty</a>
</div>
<div class="answer">
<a class="code-of-conduct" href="https://www.youtube.com/watch?v=sGQy0r_icWc" target="_blank">
<NAME> discusses the collection of 300+ hours of labeled speech corpus which enabled the creation of an automatic speech recognizer using mozilla’s deepspeech. they now use it to accelerate the transcription of native speaker archives, using tech to create systems that preserve indigenous languages.
</a>
</div>
</div>
{/* whose land */}
<div class="qanda">
<div class="question">
<a class="code-of-conduct" href="https://www.whose.land/en/" target="_blank">whose land</a>
</div>
<div class="answer">
<a class="code-of-conduct" href="https://www.whose.land/en/" target="_blank">
since we're all going to be in different places for qwer hacks this year, take a moment to figure out and acknowledge the land you're on:)
</a>
</div>
</div>
{/* queer vocab in asl */}
<div class="qanda">
<div class="question">
<a class="code-of-conduct" href="https://www.youtube.com/watch?v=8HX0HGa-pok" target="blank">them | queer vocab in asl</a>
</div>
<div class="answer">
<a class="code-of-conduct" href="https://www.youtube.com/watch?v=8HX0HGa-pok" target="blank">
in this video, nyle dimarco and chella man walk through the essentials of queer vocabulary in american sign language and the variety of ways to communicate!
</a>
</div>
</div>
</div>
);
}
export default Resources;<file_sep>/src/components/Menu.js
import React, { useState } from "react";
import Navbar from "./Navbar";
function Menu() {
const [showNav, setshowNav] = useState(false);
function handleClick(e) {
setshowNav(!showNav);
console.log("showNav is now " + showNav);
}
return (
<div class="header">
<div class="menu" id="hamburger" onClick={handleClick}>
<div class="line"></div>
<div class="line"></div>
<div class="line"></div>
</div>
<div class="something">
<div class="head">QWER HACKS</div>
</div>
{ showNav && <div className="mobile-nav" onClick={handleClick}> <Navbar /></div >}
</div >
);
}
export default Menu;<file_sep>/src/index.js
import React from "react";
import ReactDOM from "react-dom";
import './index.css';
import { BrowserRouter, Route, Switch } from "react-router-dom";
import Navbar from "./components/Navbar";
import Footer from "./components/Footer";
import Menu from "./components/Menu";
import Home from "./components/Home";
import Apply from "./components/Apply";
import Faq from "./components/Faq";
import Schedule from "./components/Schedule";
import Sponsors from "./components/Sponsors";
import Mentors from "./components/Mentors";
import Resources from "./components/Resources";
const rootElement = document.getElementById("root");
ReactDOM.render(
<BrowserRouter>
<div>
<Menu/>
</div>
<div className="content">
<div className="desktop-nav"><Navbar/></div>
<div className="container">
<Switch>
<Route exact path="/" component={Home} />
<Route path="/apply" component={Apply} />
<Route path="/faq" component={Faq} />
<Route path="/schedule" component={Schedule} />
<Route path="/sponsors" component={Sponsors} />
<Route path="/mentors" component={Mentors} />
<Route path="/resources" component={Resources} />
</Switch>
</div>
</div>
<div>
<Footer/>
</div>
</BrowserRouter>
,
rootElement
);<file_sep>/src/components/Sponsors.js
import React from "react";
function Sponsors(props) {
return (
<div>
<img src={require("../img/mlh-trust-badge-2021-white.png")} alt="mlh badge" class="mlh" width="120px"/>
<h1><div>SPONSORS</div>
<div class="subtitle">//our <a class="edit" href="https://drive.google.com/file/d/17rk7LLz3M1pvERu4jV5smDqKyXVDLIkI/view?usp=sharing" target="_blank">sponsorship deck</a> + <a class="edit" href="https://tinyurl.com/qwer-sponsor" target="_blank">sponsorship form</a></div>
</h1>
{/* <p><b>contact us by email, facebook, or instagram if you're interested in sponsoring qwer hacks 2021!</b></p> */}
<div class="sponsor-class">
<b>#include <spirit tier></b>
<div class="sponsors">
<a class="spons-img" href="https://www.xilinx.com/" target="_blank"> <img src={require("../img/xilinx.png")} alt="xilinx logo" width="90px"/> </a>
</div>
</div>
<div class="sponsor-class">
<b>#include <harmony tier></b>
<div class="sponsors">
<a class="spons-img" href="https://www.att.com" target="_blank"><img src={require("../img/att.png")} alt="att logo" width="90px"/></a>
<a class="spons-img" href="https://robinhood.com/us/en/" target="_blank"><img src={require("../img/robinhood2.png")} alt="robinhood logo" width="90px"/></a>
</div>
</div>
<div class="sponsor-class">
<b>#include <life tier></b>
<div class="sponsors">
<a class="spons-img" href="https://www.facebook.com/" target="_blank"><img src={require("../img/facebook.png")} alt="facebook logo" width="90px"/></a>
<a class="spons-img" href="https://www.northropgrumman.com/" target="_blank"><img src={require("../img/northrop.png")} alt="northop logo" width="90px"/></a>
<a class="spons-img" href="https://www.pimco.com" target="_blank"><img src={require("../img/pimco.png")} alt="pimco logo" width="90px"/></a>
<a class="spons-img" href="https://www.fmglobal.com/" target="_blank"><img src={require("../img/fmglobal.png")} alt="fmglobal logo" width="90px"/></a>
<a class="spons-img" href="https://aerospace.org/" target="_blank"><img src={require("../img/aerospace.png")} alt="aerospace logo" width="90px"/></a>
<a class="spons-img" href="https://www.chevron.com/" target="_blank"><img src={require("../img/chevron.png")} alt="chervon logo" width="90px"/></a>
<a class="spons-img" href="https://www.marathonpetroleum.com/" target="_blank"><img src={require("../img/marathon.png")} alt="marathon logo" width="90px"/></a>
<a class="spons-img" href="http://hackp.ac/mlh-stickermule-hackathons" target="_blank"><img src={require("../img/stickermule2.png")} alt="stickermule logo" width="90px"/></a>
</div>
</div>
<h1>PARTNERS</h1>
<div class="sponsor-class">
<div class="sponsors">
<a class="spons-img" href="https://www.swe.ucla.edu/" target="_blank"><img src={require("../img/swe.png")} alt="swe-ucla logo" width="100px"/></a>
<a class="spons-img" href="https://teachla.uclaacm.com" target="_blank"><img src={require("../img/acm-teachla-updated.png")} alt="teach LA logo" width="90px"/></a>
<a class="spons-img" href="https://w.uclaacm.com" target="_blank"><img src={require("../img/acm-w-updated.png")} alt="acm-w logo" width="90px"/></a>
<a class="spons-img" href="https://www.instagram.com/queersinstem/" target="_blank"><img src={require("../img/qtstem.png")} alt="acm-w logo" width="90px"/></a>
<a class="spons-img" href="http://bruinentrepreneurs.org/" target="_blank"><img src={require("../img/be.png")} alt="be logo" width="90px"/></a>
<a class="spons-img" href="https://www.uclaacm.com/" target="_blank"><img src={require("../img/acm-impact.png")} alt="be logo" width="100px"/></a>
<a class="spons-img" href="https://uclaacmai.github.io/" target="_blank"><img src={require("../img/acm-ai.png")} alt="be logo" width="90px"/></a>
<a class="spons-img" href="https://www.uclaallbrains.org/" target="_blank"><img src={require("../img/allbrains.png")} alt="all brains logo" width="90px"/></a>
<a class="spons-img" href="https://www.uclaacm.com/" target="_blank"><img src={require("../img/acm.png")} alt="acm logo" width="90px"/></a>
</div>
</div>
</div>
);
}
export default Sponsors;<file_sep>/src/components/Mentors.js
import React from "react";
function Mentors(props) {
return (
<div>
<img src={require("../img/mlh-trust-badge-2021-white.png")} alt="mlh badge" class="mlh" width="120px" />
<h1>MENTORS
<div class="subtitle">//<a class="edit" href="https://tinyurl.com/qwer-hacks-collab" target="blank"> interested in mentoring?</a></div>
</h1>
<div class="mentors">
<div class="people">
<div class="mentor-image">
<img src={require("../img/raksha.png")} alt="raksha" width="160px" />
</div>
<div class="mentor-text">
<div><a href="http://raksha.gay" target="blank" class="mentor-name"><NAME> (she/her/hers)</a></div>
<div class="mentor-info">Raksha is a software engineer at Google by day and community organizer by night. she is passionate about building a tech future for the many, not just the few. Raksha organizes with DSA Tech Action, Manhattan Community Board 4, and through freelance writing.
ask Raksha about <b>Java, Typescript/Javascript, technical communication, webdev, data structures, resume feedback, and git(hub)!</b>
</div>
</div>
</div>
<div class="people">
<div class="mentor-image">
<img src={require("../img/moss.png")} alt="moss" width="160px" />
</div>
<div class="mentor-text">
<div><a href="https://www.linkedin.com/in/annegail-moreland" target="blank" class="mentor-name"><NAME> (they/them/theirs)</a></div>
<div class="mentor-info">Moss is a software engineer at Travelers Insurance. they
recently graduated as a Cognitive Computer Science Major and are passionate
about solving complex problems for the greater benefit of humankind.
they are perpetually working towards learning new skills, finding the best ways to teach others,
and building the future, ethics first. ask Moss about <b> machine learning, NLP, and algorithmic bias!</b>
</div>
</div>
</div>
<div class="people">
<div class="mentor-image">
<img src={require("../img/lucas.jpg")} alt="lucas" width="160px" />
</div>
<div class="mentor-text">
<div><a href="https://soldaini.net" target="blank" class="mentor-name"><NAME> (he/him/his)</a></div>
<div class="mentor-info">Luca is an applied scientist at Amazon Alexa working on question-answering models.
his team builds algorithms that answer users' questions by searching on the web. he identifies as queer and pan.
ask Luca about
<b> machine learning, NLP, and artificial intelligence!</b> </div>
</div>
</div>
{/* <div class="people">
<div class="mentor-image">
<img src={require("../img/christine.png")} alt="christine" width="160px" />
</div>
<div class="mentor-text">
<div><a href="https://www.linkedin.com/in/christine-keech-026b81173/" target="blank" class="mentor-name"><NAME> (she/her/hers)</a></div>
<div class="mentor-info">I am a student living a semi-false life in a conservative area.
I do this to protect myself but I want to change this! I have been working to find others in my community to meet and bond with.
</div>
</div>
</div> */}
<div class="people">
<div class="mentor-image">
<img src={require("../img/lynn.jfif")} alt="lynn" width="160px" />
</div>
<div class="mentor-text">
<div><a href="https://www.linkedin.com/in/lynnmatar/" target="blank" class="mentor-name"><NAME> (she/her/hers)</a></div>
<div class="mentor-info">Lynn is a Lebanese Bisexual Computer Science student at Illinois Tech that wants to spread awareness about mental health and help the LGBTQ+ community.
ask Lynn about
<b> Python, Java, debugging, and github!</b></div>
</div>
</div>
<div class="people">
<div class="mentor-image">
<img src={require("../img/aru.png")} alt="aru" width="160px" />
</div>
<div class="mentor-text">
<div><a href="https://www.linkedin.com/in/aru-bhola/" target="blank" class="mentor-name"><NAME> (she/her/hers)</a></div>
<div class="mentor-info">Aru is a second year computer engineering student who just wants to build robots. ask Aru about
<b> C/C++ and Hardware Design Language!</b></div>
</div>
</div>
<div class="people">
<div class="mentor-image">
<img src={require("../img/yogendra.jfif")} alt="yogendra" width="160px" />
</div>
<div class="mentor-text">
<div><a href="https://studentambassadors.microsoft.com/en-US/profile/4146" target="blank" class="mentor-name"><NAME> (he/him/his)</a></div>
<div class="mentor-info"> Yogendrasingh is a student in Mumbai, India at NMIMS University.
he has mentored at several hackathons
and loves seeing people grow and learn in such a short span of time.
ask Yogendrasingh about
<b> web development, AI/ML, and debugging!</b></div>
</div>
</div>
<div class="people">
<div class="mentor-image">
<img src={require("../img/martin.jfif")} alt="martin" width="160px" />
</div>
<div class="mentor-text">
<div><a href="https://www.linkedin.com/in/martin-stoyanov-745b4272/" target="blank" class="mentor-name"><NAME> (he/him/his)</a></div>
<div class="mentor-info"> Martin is the co-founder of Emailio, an iOS developer, Thiel Fellowship recipient, and the youngest founder to graduate from Y Combinator.
ask Martin about <b> React, iOS development, and turning your project into a startup!</b></div>
</div>
</div>
<div class="people">
<div class="mentor-image">
<img src={require("../img/matt.png")} alt="facebook logo" width="160px"/>
</div>
<div class="mentor-text">
<div><a href="https://matthewwang.me" target="blank" class="mentor-name"><NAME> (he/him/his)</a></div>
<div class="mentor-info">Matt is a third-year CS + Math-Econ student at UCLA who's passionate about using computers to make the world a fairer place.
He spends most of his time at ACM Teach LA, trying to make computer science education more accessible!
ask Matt about <b> HTML/CSS/JS, MV frameworks (React, Angular, etc), general frontend/backend design, and deploying an application + server admin</b>
</div>
</div>
</div>
<div class="people">
<div class="mentor-image">
<img src={require("../img/ariel_z.png")} alt="facebook logo" width="160px"/>
</div>
<div class="mentor-text">
<div><a href="https://www.arielzucker.com" target="blank" class="mentor-name"><NAME> (she/her/hers)</a></div>
<div class="mentor-info"><NAME> is the founder and co-CEO of CNT Productions, where she directs and produces socially charged content with a bite.
She is also the Director of Digital Live Production at YEA!
Impact where she coordinates virtual live events.
Her work has been recognized by Outfest and NBC Out, amongst others, and her virtual events have
included collaborations with Netflix, Disney+, Hovercast, and more. Ask Ariel about
<b> creative collaboration, impact producing, virtual live broadcasting.</b>
</div>
</div>
</div>
</div>
</div>
);
}
export default Mentors;<file_sep>/README.md
# Wrong Repository
see https://github.com/qwerhacks/QWERHACKS-website-2022
<file_sep>/src/components/Navbar.js
import "../index.css";
import React from "react";
import {NavLink } from "react-router-dom";
function Navbar() {
return (
<div class="nav" id="nbar">
<div class="buttons">
<NavLink exact className = "text-link" to="/"><button type="button" id="about" class="b">home</button></NavLink>
<NavLink className = "text-link" to="/apply"><button type="button" id="faq" class="b">apply!</button></NavLink>
<NavLink className = "text-link" to="/faq"><button type="button" id="sched" class="b">faq</button></NavLink>
<NavLink className = "text-link" to="/schedule"><button type="button" id="sandp" class="b">schedule</button></NavLink>
<NavLink className = "text-link" to="/sponsors"><button type="button" id="resources" class="b">sponsors</button></NavLink>
<NavLink className = "text-link" to="/mentors"><button type="button" id="resources" class="b">mentors</button></NavLink>
<NavLink className = "text-link" to="/resources"><button type="button" id="resources" class="b">resources</button></NavLink>
</div>
<div class="social_media">
<div class="icon">
<a href="https://www.facebook.com/qwerhacks/" target="_blank"><img src={require("../img/fb-icon.png")} alt="facebook logo" width="30px"/></a>
</div>
<div class="icon">
<a href="https://www.instagram.com/qwerhacks/" target="_blank"><img src={require("../img/ig-icon.png")} alt="instagram logo" width="30px"/></a>
</div>
<div class="icon">
<a href="mailto:<EMAIL>"><img src={require("../img/email-icon.png")} alt="email logo" width="30px"/></a>
</div>
<div class="icon">
<a href="https://twitter.com/qwerhacks" target="_blank"><img src={require("../img/icon-twitter.png")} alt="twitter logo" width="30px"/></a>
</div>
</div>
</div>
);
}
export default Navbar;
<file_sep>/src/components/Faq.js
import React from "react";
function Faq(props) {
return (
<div class="faq">
<img src={require("../img/mlh-trust-badge-2021-white.png")} alt="mlh badge" class="mlh" width="120px"/>
<h1>FAQ</h1>
<div class="qanda">
<div class="question">
who can come?
</div>
<div class="answer">
anyone who is 18 or older, a member (or ally) of the LGBTQIA+ community, and enrolled
in a postsecondary academic institution is invited to participate!
</div>
</div>
<div class="qanda">
<div class="question">
how will teams work?
</div>
<div class="answer">
you can sign up as a team of up to four members. but make sure that all
your team members submit an application! if you don’t have a team before the event,
don’t worry! we will help you find fellow hackers once the event starts :^)
</div>
</div>
<div class="qanda">
<div class="question">
what can i create?
</div>
<div class="answer">
absolutely anything you want –– websites, apps, UI, you name it!
we encourage you to create a product that positively impacts the LGBTQIA+ community or
any other underrepresented group in tech.
</div>
</div>
<div class="qanda">
<div class="question">
how much does it cost?
</div>
<div class="answer">
it's free, babey!
</div>
</div>
<div class="qanda">
<div class="question">
can allies attend?
</div>
<div class="answer">
yes! we welcome anyone who supports our mission of increasing the visibility
of our community in tech :)
</div>
</div>
<div class="qanda">
<div class="question">
sounds great, how can i apply?
</div>
<div class="answer">
go to our apply page and fill out the google form!
</div>
</div>
<div class="qanda">
<div class="question">
what is tikkl?
</div>
<div class="answer">
tikkl is a live events platform that we are using to stream qwerhacks events like speeches and workshops.
</div>
</div>
<div class="qanda">
<div class="question">
how do i attend keynote speeches?
</div>
<div class="answer">
you can watch them live on tikkl!
</div>
</div>
<div class="qanda">
<div class="question">
how do i attend workshops?
</div>
<div class="answer">
one of two ways, but both on tikkl! for streamed workshops, you can watch them in the same way you watched the keynote speeches.
for interactive workshops, a zoom link will be posted on tikkl! when it’s time for the workshop to start, click on the link and you can hop right in.
</div>
</div>
<div class="qanda">
<div class="question">
how do i talk to my team?
</div>
<div class="answer">
on our discord! once you register with your team, you’ll have your own dedicated voice and text channel on the server you can use to talk to your teammates!
</div>
</div>
<div class="qanda">
<div class="question">
how do i talk to mentors?
</div>
<div class="answer">
on our discord! we have multiple channels for mentorship questions. if you’re wondering about which specific mentors are available, you can look at the speakers tab on tikkl.
</div>
</div>
<div class="qanda">
<div class="question">
how will judging work?
</div>
<div class="answer">
you’ll submit a two minute video of your project on our devpost, and from there the judges will watch and score! there will be no live q&a portion.
</div>
</div>
<div class="qanda">
<div class="question">
what will i do while i wait for judging to happen?
</div>
<div class="answer">
vibeeeee with us. we’ll be having our third identity specific mentorship group meeting for those participating, and fun low commitment activities for those who aren’t!
</div>
</div>
</div>
);
}
export default Faq; | c5a0f769a3da8da1c4cf62bdf42c925a0aaf246c | [
"JavaScript",
"Markdown"
] | 8 | JavaScript | qwerhacks/QWERHacks2022 | 126c185b0a947abdddf35868e3753562f23ffed8 | b4e290b9734edc07a01868f9a3ce7c1377e1018e | |
refs/heads/master | <file_sep>package com.project.nra;
import android.content.Context;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.AsyncTask;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
import android.widget.ImageView;
import android.widget.TextView;
import com.project.nra.news_lib.model.News;
import java.io.InputStream;
import java.util.ArrayList;
/**
* Created by hp.luong on 3/24/2017.
*/
public class NewsAdapter extends ArrayAdapter<News> {
private ArrayList<News> newsList;
public NewsAdapter(Context context, ArrayList<News> news) {
super(context, 0, news);
this.newsList = news;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
News news = newsList.get(position);
if (convertView == null) {
convertView = LayoutInflater.from(getContext()).inflate(R.layout.activity_listview, parent, false);
}
if (news != null) {
TextView txtNews = (TextView) convertView.findViewById(R.id.txtNews);
ImageView imageView = (ImageView) convertView.findViewById(R.id.iconImage);
TextView txtScore = (TextView) convertView.findViewById(R.id.txtScore);
if (txtNews != null) {
txtNews.setText(news.getTitle());
// new DownloadImageTask(imageView).execute(news.getUrlToImage());
Bitmap bmpImage = BitmapFactory.decodeByteArray(news.getImage(), 0, news.getImage().length);
imageView.setImageBitmap(bmpImage);
txtScore.setText("1.0");
}
}
return convertView;
}
}
class DownloadImageTask extends AsyncTask<String, Void, Bitmap> {
ImageView bmImage;
public DownloadImageTask(ImageView bmImage) {
this.bmImage = bmImage;
}
protected Bitmap doInBackground(String... urls) {
String urldisplay = urls[0];
Bitmap mIcon11 = null;
try {
InputStream in = new java.net.URL(urldisplay).openStream();
mIcon11 = BitmapFactory.decodeStream(in);
} catch (Exception e) {
Log.d("Error", e.getMessage());
e.printStackTrace();
}
return mIcon11;
}
protected void onPostExecute(Bitmap result) {
bmImage.setImageBitmap(result);
}
}
<file_sep>import numpy as np # linear algebra
import pandas as pd # data processing, CSV file I/O (e.g. pd.read_csv)
import matplotlib.pyplot as plt
from matplotlib import pyplot
from mpl_toolkits.mplot3d import Axes3D
from sklearn.cluster import KMeans
import matplotlib.axes as ax
data = pd.read_csv("new_data3.csv")
data.shape
print (data.head())
#replace negative values
data['sum'][data['sum']<0] = data['sum'][data['sum']<0]*(-1)
#print (data.head())
#for 2D, needs 2 data columns
X = data.iloc[:,2:4].values
#for 3D, needs 3 data columns
#X = data.iloc[:,1:4].values
print(X.shape)
X_1 = data[data.columns[2]]
X_2 = data[data.columns[3]]
print (X_1.min(), X_1.max())
print (X_2.min(), X_2.max())
k = 3
kmeans = KMeans(n_clusters=k)
kmeans.fit(X)
labels = kmeans.labels_
print("labels:", labels)
centroids = kmeans.cluster_centers_
print("centroids:", centroids)
for i in range(k):
# select only data observations with cluster label == i
ds = X[np.where(labels==i)]
#
# plot the data observations
pyplot.plot(ds[:,0],ds[:,1],'o')
# plot the centroids
lines = pyplot.plot(centroids[i,0],centroids[i,1],'x')
# make the centroid x's bigger
# pyplot.setp(lines,ms=15.0)
# pyplot.setp(lines,mew=2.0)
pyplot.xlim([X_1.min(),X_1.max()])
pyplot.ylim([X_2.min(),X_2.max()])
# fig = pyplot.figure()
# fig.suptitle('bold figure suptitle', fontsize=14, fontweight='bold')
# ax = fig.add_subplot(111)
# fig.subplots_adjust(top=0.85)
# ax.set_title('axes title')
# ax.set_xlabel('xlabel')
# ax.set_ylabel('ylabel')
pyplot.xlabel('num_session')
pyplot.ylabel('total_time')
#pyplot.xrange.ticklabel_format(style='plain')
#ax.Axes.get_yaxis().get_major_formatter().set_useOffset(False)
pyplot.show()
# fig = plt.figure(1, figsize=(4, 3))
# ax = Axes3D(fig, rect=[0, 0, .95, 1], elev=48, azim=134)
# kmeans.fit(X)
# labels = kmeans.labels_
# ax.scatter(X[:, 0], X[:, 1], X[:, 2],
# c=labels.astype(np.float), edgecolor='k')
# ax.w_xaxis.set_ticklabels([])
# ax.w_yaxis.set_ticklabels([])
# ax.w_zaxis.set_ticklabels([])
# ax.set_xlabel('num_apps')
# ax.set_ylabel('num_session')
# ax.set_zlabel('total_time')
# ax.dist = 12
# plt.show()<file_sep>package com.example.testapp;
import java.io.UnsupportedEncodingException;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import android.app.enterprise.ApplicationPolicy;
import android.app.enterprise.EnterpriseDeviceManager;
import android.content.Context;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.util.Base64;
import android.util.Log;
public class EdmPolicyUtils {
private static final String LOG_TAG = "EdmPolicyUtils";
public static void startApplication(Context ctxt, String packageName) {
PackageManager pm = ctxt.getPackageManager();
Intent launch = pm.getLaunchIntentForPackage(packageName);
if (launch == null) {
Log.w(LOG_TAG, packageName + " is not installed");
} else {
launch.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
ctxt.startActivity(launch);
}
}
public static boolean stopApplication(Context ctxt, String packageName) {
EnterpriseDeviceManager edm = (EnterpriseDeviceManager) ctxt
.getSystemService(EnterpriseDeviceManager.ENTERPRISE_POLICY_SERVICE);
ApplicationPolicy appPolicy = edm.getApplicationPolicy();
return appPolicy.stopApp(packageName);
}
public static String SHA1(String text)
throws NoSuchAlgorithmException, UnsupportedEncodingException {
MessageDigest md;
md = MessageDigest.getInstance("SHA-1");
byte[] sha1hash = new byte[40];
md.update(text.getBytes("iso-8859-1"), 0, text.length());
sha1hash = md.digest();
String digestStr =Base64.encodeToString(sha1hash,0);
return digestStr;
}
public static boolean verifyDigest( String userKey, String inDigest ) throws Exception{
boolean ret = false;
if (userKey == null || inDigest == null ){
return ret;
}
String outDigest = SHA1 (userKey);
Log.d(LOG_TAG, "Digest: " + " inDigest: #" + inDigest + "# outDigest: #" + outDigest +"#");
if (outDigest.equals(inDigest)){
Log.d(LOG_TAG, " Digests are equal");
ret = true;
}else {
Log.d(LOG_TAG," Digests are not equal");
}
return ret;
}
}
<file_sep>import numpy as np
import matplotlib.pyplot as plt
from scipy.spatial.distance import cdist
from sklearn.cluster import KMeans
from sklearn.decomposition import PCA
#N = 500
N = 1000
X = np.loadtxt("data1.txt", dtype = (float, float), delimiter='\t', usecols=(4,5))
print (X.shape)
#print(X[:,0])
plt.hist(X[:,1])
#plt.show()
kmeans_model = KMeans(n_clusters=3, random_state=1)
kmeans_model.fit(X)
labels = kmeans_model.labels_
#print(labels)
pca_2 = PCA(2)
plot_columns = pca_2.fit_transform(X)
plt.scatter(x=plot_columns[:,0], y=plot_columns[:,1], c=labels)
plt.show()
<file_sep>package com.project.nra.news_lib.model;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
/**
* Created by hp.luong on 7/7/2016.
*/
public class JsonUtils {
public static String getJsonResult(String webURL) throws MalformedURLException {
StringBuilder result = new StringBuilder();
HttpURLConnection connection = null;
URL url = new URL(webURL);
try {
connection = (HttpURLConnection) url.openConnection();
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String inputLine;
while ((inputLine = in.readLine()) != null) {
result.append(inputLine);
}
in.close();
} catch (IOException e) {
System.out.println("IOException: " + e.toString());
} catch (Exception e) {
System.out.println("Exception: " + e.toString());
} finally {
if (connection != null)
connection.disconnect();
}
return result.toString();
}
}
<file_sep>def twoSum(list_num, target):
arr = {}
for idx, item in enumerate(list_num):
complement = target - item
if (complement in arr):
return
<file_sep>def bubble_sort(array):
for i in range(len(array) - 1, 0, -1):
for j in range(0, i):
if (array[j + 1] < array[j]):
swap(array, j, j+1)
print("swapped: i = %s j = %s" %(i,j),array)
return array
def bubble_sort2(array):
for i in range(0, len(array) - 1):
for j in range(0, len(array) -1 - i):
if (array[j + 1] < array[j]):
swap(array, j, j+1)
print("swapped: i = %s j = %s" %(i,j),array)
return array
def swap(array, i, j):
tmp = array[i]
array[i] = array[j]
array[j] = tmp
unsorted = [2,4,1,5,9,7,3]
print("Before: ", unsorted)
sorted = bubble_sort2(unsorted)
print("After: ", sorted)
<file_sep>import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
import matplotlib.pyplot as plt
#matplotlib inline
data_train = pd.read_csv('train.csv')
data_test = pd.read_csv('test.csv')
#print (data_train.describe())
#print (data_train[['Pclass', 'Survived']].groupby(['Pclass'], as_index=False).mean())
#print(pd.crosstab(data_train['Pclass'], data_train['Survived']))
#print(data_test.describe())
#sns.barplot(x="Embarked", y="Survived", hue="Sex", data=data_train);
#plt.show();
print(data_train.head(5))
<file_sep>class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def addToFront(self, new_data):
newNode = Node(new_data)
newNode.next = self.head
self.head = newNode
def addToTail(self, new_data):
newNode = Node(new_data)
temp = self.head
if (temp == None):
self.head = newNode
else:
while (temp.next != None):
temp = temp.next
temp.next = newNode
def deleteNode(self, node_data):
found = False
current = self.head
if (current == None):
return
elif (current.data == node_data):
if (current.next == None):
self.head = None
else:
self.head = current.next
else:
while (current.next != None):
prev = current
current = current.next
if (current.data == node_data):
prev.next = current.next
print ("Node found and deleted")
found = True
if (not found):
print("Node does not exist")
def printList(self):
temp = self.head
while(temp):
print (temp.data)
temp = temp.next
#MAIN RUN
if __name__=='__main__':
lst = LinkedList()
lst.head = Node(1)
second = Node(2)
third = Node(3)
lst.head.next = second
second.next = third
lst.printList()
print("Add node 5 to the head of the list")
lst.addToFront(5)
lst.printList()
print("Add node to the end of the list")
lst.addToTail(8)
lst.printList()
print("Delete node")
lst.deleteNode(5)
lst.deleteNode(8)
lst.deleteNode(8)
lst.printList()
<file_sep>def fibonacci(n):
if n == 0: return 0
if n == 1: return 1
return fibonacci(n-1) + fibonacci(n-2)
ret = []
def fibonacci2(n):
if n == 0:
ret.append(0)
return ret
if n == 1:
ret.append(1)
return ret
tmp = fibonacci2(n-1) + fibonacci2(n-2)
ret.append(tmp)
return ret
cache = []
def fibonacci_ds(n):
if n == 0: cache.insert(0,0)
if n == 1: cache.insert(1,1)
if n > 1: cache.insert(n,fibonacci_ds(n-1) + fibonacci_ds(n-2))
return cache[n]
print (fibonacci(9))
#print (fibonacci_ds(9))
print(fibonacci2(3))<file_sep>include ':MainApp'
include ':LIBRARY:mylibrary1'
include ':LIBRARY:mylibrary2'<file_sep>package com.project.nra.news_lib.model;
import android.graphics.Bitmap;
import java.util.ArrayList;
public class News {
private int id;
private String source;
private String author;
private String title;
private String description;
private String url;
private String urlToImage;
private String publishedAt;
private byte[] image;
public News(int id, String source, String author, String title, String description, String url, String urlToImage, String publishedAt, byte[] image) {
this.id = id;
this.source = source;
this.author = author;
this.title = title;
this.description = description;
this.url = url;
this.urlToImage = urlToImage;
this.publishedAt = publishedAt;
this.image = image;
}
public News() {
this.id = 0;
this.source = null;
this.author = null;
this.description = null;
this.title = null;
this.url = null;
this.urlToImage = null;
this.publishedAt = null;
}
@Override
public String toString() {
return getTitle();
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public String getUrlToImage() {
return urlToImage;
}
public void setUrlToImage(String urlToImage) {
this.urlToImage = urlToImage;
}
public String getPublishedAt() {
return publishedAt;
}
public void setPublishedAt(String publishedAt) {
this.publishedAt = publishedAt;
}
public String getSource() {
return source;
}
public void setSource(String source) {
this.source = source;
}
public byte[] getImage() {
return image;
}
public void setImage(byte[] image) {
this.image = image;
}
}
<file_sep>package com.project.nra.news_lib.db;
import android.content.ContentValues;
import android.content.Context;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import android.util.Log;
import com.project.nra.news_lib.model.News;
import java.io.File;
import java.util.ArrayList;
import java.util.List;
/**
* Created by hp.luong on 3/8/2017.
*/
public class DBHelper extends SQLiteOpenHelper {
private static final String TAG = DBHelper.class.getSimpleName();
private static final int DATABASE_VERSION = 1;
private static final String DATABASE_NAME = "news.db";
private static final String TABLE_NEWS = "news";
private static final String COLUMN_NEWS_ID = "id";
private static final String COLUMN_NEWS_SOURCE = "source";
private static final String COLUMN_NEWS_AUTHOR = "author";
private static final String COLUMN_NEWS_TITLE = "title";
private static final String COLUMN_NEWS_DESC = "description";
private static final String COLUMN_NEWS_URL = "url";
private static final String COLUMN_NEWS_URLTOIMAGE = "url_to_image";
private static final String COLUMN_NEWS_PUBLISHEDAT = "published_at";
private static final String COLUMN_NEWS_IMAGE = "news_image";
private static final int INDEX_COLUMN_NEWS_ID = 0;
private static final int INDEX_COLUMN_NEWS_SOURCE = 1;
private static final int INDEX_COLUMN_NEWS_AUTHOR = 2;
private static final int INDEX_COLUMN_NEWS_TITLE = 3;
private static final int INDEX_COLUMN_NEWS_DESC = 4;
private static final int INDEX_COLUMN_NEWS_URL = 5;
private static final int INDEX_COLUMN_NEWS_URLTOIMAGE = 6;
private static final int INDEX_COLUMN_NEWS_PUBLISHEDAT = 7;
private static final int INDEX_COLUMN_NEWS_IMAGE = 8;
public static DBHelper dbHelper;
public DBHelper(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
}
public static synchronized DBHelper getInstance(Context context) {
if (dbHelper == null) {
dbHelper = new DBHelper(context);
}
return dbHelper;
}
@Override
public void onCreate(SQLiteDatabase db) {
Log.d(TAG, "onCreate");
String CREATE_NEWS_TABLE = "CREATE TABLE " + TABLE_NEWS + "("
+ COLUMN_NEWS_ID + " INTEGER PRIMARY KEY,"
+ COLUMN_NEWS_SOURCE + " TEXT,"
+ COLUMN_NEWS_AUTHOR + " TEXT,"
+ COLUMN_NEWS_TITLE + " TEXT,"
+ COLUMN_NEWS_DESC + " TEXT,"
+ COLUMN_NEWS_URL + " TEXT,"
+ COLUMN_NEWS_URLTOIMAGE + " TEXT,"
+ COLUMN_NEWS_PUBLISHEDAT + " TEXT,"
+ COLUMN_NEWS_IMAGE + " BLOB" + ");";
db.execSQL(CREATE_NEWS_TABLE);
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
// Drop older table if existed
db.execSQL("DROP TABLE IF EXISTS " + TABLE_NEWS);
// Create tables again
onCreate(db);
}
public void addNews(News news) {
SQLiteDatabase db = this.getWritableDatabase();
ContentValues values = new ContentValues();
values.put(COLUMN_NEWS_ID, news.getId());
values.put(COLUMN_NEWS_SOURCE, news.getSource());
values.put(COLUMN_NEWS_AUTHOR, news.getAuthor());
values.put(COLUMN_NEWS_TITLE, news.getTitle());
values.put(COLUMN_NEWS_DESC, news.getDescription());
values.put(COLUMN_NEWS_URL, news.getUrl());
values.put(COLUMN_NEWS_URLTOIMAGE, news.getUrlToImage());
values.put(COLUMN_NEWS_PUBLISHEDAT, news.getPublishedAt());
values.put(COLUMN_NEWS_IMAGE, news.getImage());
db.insert(TABLE_NEWS, null, values);
db.close();
}
public boolean isNewsExisted(String url) {
SQLiteDatabase db = this.getReadableDatabase();
Cursor cursor = db.query(TABLE_NEWS, new String[] { COLUMN_NEWS_ID,
COLUMN_NEWS_SOURCE, COLUMN_NEWS_AUTHOR, COLUMN_NEWS_TITLE, COLUMN_NEWS_DESC, COLUMN_NEWS_URL,
COLUMN_NEWS_URLTOIMAGE, COLUMN_NEWS_PUBLISHEDAT, COLUMN_NEWS_IMAGE}, COLUMN_NEWS_URL + "=?",
new String[] {url}, null, null, null, null);
if (cursor != null && cursor.moveToFirst()) {
return true;
}
return false;
}
public News getNews(int newsId) {
SQLiteDatabase db = this.getReadableDatabase();
Cursor cursor = db.query(TABLE_NEWS, new String[] { COLUMN_NEWS_ID,
COLUMN_NEWS_SOURCE, COLUMN_NEWS_AUTHOR, COLUMN_NEWS_TITLE, COLUMN_NEWS_DESC, COLUMN_NEWS_URL,
COLUMN_NEWS_URLTOIMAGE, COLUMN_NEWS_PUBLISHEDAT, COLUMN_NEWS_IMAGE}, COLUMN_NEWS_ID + "=?",
new String[] { String.valueOf(newsId) }, null, null, null, null);
if (cursor != null && cursor.moveToFirst()) {
News news = new News(Integer.parseInt(cursor.getString(INDEX_COLUMN_NEWS_ID)),
cursor.getString(INDEX_COLUMN_NEWS_SOURCE), cursor.getString(INDEX_COLUMN_NEWS_AUTHOR), cursor.getString(INDEX_COLUMN_NEWS_TITLE), cursor.getString(INDEX_COLUMN_NEWS_DESC),
cursor.getString(INDEX_COLUMN_NEWS_URL), cursor.getString(INDEX_COLUMN_NEWS_URLTOIMAGE), cursor.getString(INDEX_COLUMN_NEWS_PUBLISHEDAT), cursor.getBlob(INDEX_COLUMN_NEWS_IMAGE));
return news;
}
return null;
}
public List<News> getAllNews() {
List<News> newsList = new ArrayList<News>();
String selectQuery = "SELECT * FROM " + TABLE_NEWS;
SQLiteDatabase db = this.getWritableDatabase();
Cursor cursor = db.rawQuery(selectQuery, null);
if (cursor.moveToFirst()) {
do {
News news = new News();
news.setId(Integer.parseInt(cursor.getString(INDEX_COLUMN_NEWS_ID)));
news.setSource(cursor.getString(INDEX_COLUMN_NEWS_SOURCE));
news.setAuthor(cursor.getString(INDEX_COLUMN_NEWS_AUTHOR));
news.setTitle(cursor.getString(INDEX_COLUMN_NEWS_TITLE));
news.setDescription(cursor.getString(INDEX_COLUMN_NEWS_DESC));
news.setUrl(cursor.getString(INDEX_COLUMN_NEWS_URL));
news.setUrlToImage(cursor.getString(INDEX_COLUMN_NEWS_URLTOIMAGE));
news.setPublishedAt(cursor.getString(INDEX_COLUMN_NEWS_PUBLISHEDAT));
news.setImage(cursor.getBlob(INDEX_COLUMN_NEWS_IMAGE));
newsList.add(news);
} while (cursor.moveToNext());
}
return newsList;
}
public ArrayList<String> getNewsTitleBySource(String source) {
ArrayList<String> newsTitleList = new ArrayList<String>();
List<News> newsList = getNewsBySource(source);
if (newsList.size() > 0) {
for (News news: newsList) {
newsTitleList.add(news.getTitle());
}
}
return newsTitleList;
}
public ArrayList<String> getNewsSources() {
Log.d(TAG, "getNewsSources... news count = " + getNewsCount());
ArrayList<String> newsSourceList = new ArrayList<String>();
SQLiteDatabase db = this.getReadableDatabase();
String countQuery = "SELECT DISTINCT source FROM " + TABLE_NEWS + ";";
Cursor cursor = db.rawQuery(countQuery, null);
if (cursor.moveToFirst()) {
do {
newsSourceList.add(cursor.getString(0));
} while (cursor.moveToNext());
}
Log.d(TAG, "newsSourceList = " + newsSourceList.size());
return newsSourceList;
}
public List<News> getNewsBySource(String source) {
List<News> newsList = new ArrayList<News>();
SQLiteDatabase db = this.getWritableDatabase();
Cursor cursor = db.query(TABLE_NEWS, new String[] { COLUMN_NEWS_ID,
COLUMN_NEWS_SOURCE, COLUMN_NEWS_AUTHOR, COLUMN_NEWS_TITLE, COLUMN_NEWS_DESC, COLUMN_NEWS_URL,
COLUMN_NEWS_URLTOIMAGE, COLUMN_NEWS_PUBLISHEDAT, COLUMN_NEWS_IMAGE}, COLUMN_NEWS_SOURCE + "=?",
new String[] {source}, null, null, null, null);
if (cursor.moveToFirst()) {
do {
News news = new News();
news.setId(Integer.parseInt(cursor.getString(INDEX_COLUMN_NEWS_ID)));
news.setSource(cursor.getString(INDEX_COLUMN_NEWS_SOURCE));
news.setAuthor(cursor.getString(INDEX_COLUMN_NEWS_AUTHOR));
news.setTitle(cursor.getString(INDEX_COLUMN_NEWS_TITLE));
news.setDescription(cursor.getString(INDEX_COLUMN_NEWS_DESC));
news.setUrl(cursor.getString(INDEX_COLUMN_NEWS_URL));
news.setUrlToImage(cursor.getString(INDEX_COLUMN_NEWS_URLTOIMAGE));
news.setPublishedAt(cursor.getString(INDEX_COLUMN_NEWS_PUBLISHEDAT));
news.setImage(cursor.getBlob(INDEX_COLUMN_NEWS_IMAGE));
newsList.add(news);
} while (cursor.moveToNext());
}
return newsList;
}
public void removeNews(News news) {
SQLiteDatabase db = this.getWritableDatabase();
db.delete(TABLE_NEWS, COLUMN_NEWS_ID + " = ?",
new String[] { String.valueOf(news.getId()) });
db.close();
}
public int updateNews (News news) {
SQLiteDatabase db = this.getWritableDatabase();
ContentValues values = new ContentValues();
values.put(COLUMN_NEWS_ID, news.getId());
values.put(COLUMN_NEWS_SOURCE, news.getSource());
values.put(COLUMN_NEWS_AUTHOR, news.getAuthor());
values.put(COLUMN_NEWS_TITLE, news.getTitle());
values.put(COLUMN_NEWS_DESC, news.getDescription());
values.put(COLUMN_NEWS_URL, news.getUrl());
values.put(COLUMN_NEWS_URLTOIMAGE, news.getUrlToImage());
values.put(COLUMN_NEWS_PUBLISHEDAT, news.getPublishedAt());
values.put(COLUMN_NEWS_IMAGE, news.getImage());
return db.update(TABLE_NEWS, values, COLUMN_NEWS_ID + " = ?",
new String[] { String.valueOf(news.getId()) });
}
public int getNewsCount() {
String countQuery = "SELECT * FROM " + TABLE_NEWS + ";";
SQLiteDatabase db = this.getReadableDatabase();
Cursor cursor = db.rawQuery(countQuery, null);
int count = cursor.getCount();
cursor.close();
return count;
}
public long getDBSize() {
SQLiteDatabase db = this.getReadableDatabase();
return new File(db.getPath()).length();
}
}
<file_sep>package com.project.nra.news_lib.model;
/**
* Created by hp.luong on 7/7/2016.
*/
public class Constants {
public static final String STATUS = "status";
public static final String STATUS_OK = "ok";
public static final String STATUS_ERROR = "error";
public static final String SOURCES = "sources";
public static final String SOURCE_ID = "id";
public static final String ARTICLES = "articles";
public static final String AUTHOR = "author";
public static final String DESCRIPTION = "description";
public static final String TITLE = "title";
public static final String URL = "url";
public static final String URL_IMAGE = "urlToImage";
public static final String PUBLISHED_AT = "publishedAt";
public static final String API_KEY = "de2f02ca31e54052bf41b1ae7daceafa";
public static final String SOURCE_QUERY = "https://newsapi.org/v1/sources";
public static final String PREFIX_SOURCE_QUERY ="https://newsapi.org/v1/articles?source=";
}
<file_sep>import numpy as np
data = np.loadtxt("data.txt", dtype = (int, int), delimiter='\t', usecols=(4,5))
print ("data = ", data)
<file_sep>from sys import argv
import numpy as np
def loadData(filename):
#script, filename = argv
with open(filename) as f:
lines = f.readlines()
print lines
def loadDataset(training_data, test_data):
training = np.loadtxt(training_data, dtype = 'str', delimiter = ', ', skiprows = 0)
print training.shape
print training.item((0, 1))
print training.item((2, 14))
for (row in training)
print row[0]
def main():
print "Hello"
loadDataset('adult.data', 'adult.test')
main()
<file_sep>def quicksort(array):
less = []
equal = []
greater = []
if (len(array) > 1):
pivot = array[0]
for x in array:
if (x < pivot):
less.append(x)
elif (x == pivot):
equal.append(x)
else:
greater.append(x)
return quicksort(less) + equal + quicksort(greater)
else:
return array
def main():
input = [12,5,3,3,6,7,2,45,2]
output = quicksort(input)
print("Result: ", output)
if __name__ == "__main__":main()
<file_sep>import numpy as np
import pandas as pd
data = pd.read_csv("train.csv")
#print(data.head())
test = pd.read_csv("test.csv")
#print(test.head().T)
null_columns=data.columns[data.isnull().any()]
import matplotlib.pyplot as plt
import seaborn as sns
sns.set(font_scale=1)
pd.options.display.mpl_style = 'default'
labels = []
values = []
for col in null_columns:
labels.append(col)
values.append(data[col].isnull().sum())
ind = np.arange(len(labels))
width=0.6
fig, ax = plt.subplots(figsize=(6,5))
rects = ax.barh(ind, np.array(values), color='purple')
ax.set_yticks(ind+((width)/2.))
ax.set_yticklabels(labels, rotation='horizontal')
ax.set_xlabel("Count of missing values")
ax.set_ylabel("Column Names")
ax.set_title("Variables with missing values");
data.hist(bins=10,figsize=(9,7),grid=False);
<file_sep>include ':NRA_APP'
include ':LIBRARY:NEWS_LIB'
<file_sep>import csv
with open('iris.data', 'rt') as csvfile:
lines = csv.reader(csvfile)
print (lines)
for row in lines:
print (row)
| 3346d1f2b47502775259448c895f24859fc6ff67 | [
"Java",
"Python",
"Gradle"
] | 20 | Java | mle8109/repo1 | 5fc4b68bd6442aaccb81c40505e4e77de387ca50 | 9088c7453979c7d17b9e596ae689f844475a7aa8 | |
refs/heads/master | <repo_name>paweljankowski92/task-synapsi<file_sep>/src/components/Cypher.js
import React from 'react';
const Cypher = (props) => {
const cipherCode = 3;
function encode (char) {
const letterCode = char.charCodeAt(0);
const codedChar = String.fromCharCode(letterCode + cipherCode)
return codedChar
}
function decode (char) {
const letterCode = char.charCodeAt(0);
const codedChar = String.fromCharCode(letterCode - cipherCode)
return codedChar
}
const cypherText = [...props.text].map(char => encode(char)).join('');
const unCypherText = [...props.text].map(char => decode(char)).join('');
return (
<div>
<textarea className="textarea">
{props.textCode ? cypherText : unCypherText }
</textarea>
</div>
)
}
export default Cypher;
<file_sep>/src/components/Textarea.js
import React, { Component } from 'react';
import Cypher from './Cypher';
import './Textarea.css';
import { FontAwesomeIcon } from '@fortawesome/react-fontawesome';
import { faLock } from '@fortawesome/free-solid-svg-icons';
import { faLockOpen } from '@fortawesome/free-solid-svg-icons';
class Textarea extends Component {
state = {
text: [],
changeText: false,
textCode: false,
}
code = () => {
const textarea = document.getElementById('txtarea');
const value = textarea.value;
if(value !== ''){
this.setState({
text: value,
changeText: true,
textCode: true,
})
} else {
alert('Zaby zakodować, wpisz dowolny ciąg znaków, liter lub cyfr')
this.setState({
text: '',
changeText: false,
textCode: false,
})
}
}
uncode = () => {
const textarea = document.getElementById('txtarea');
const value = textarea.value;
if(value !== ''){
this.setState({
text: value,
changeText: true,
textCode: false,
})
} else {
alert('Aby odkodować, wklej zaszyfrowany kod')
this.setState({
text: '',
changeText: false,
})
}
}
clearArea = () => {
this.setState({
changeText: false
})
}
render() {
return (
<div>
<h2 className="title2">Now, I encourage you to see the cipher generator.</h2>
<div className="columns">
<div className="cypher">
<textarea className='textarea' onChange={this.clearArea} id="txtarea" /><br />
<div className='buttons'>
<button className='btn' onClick={this.code}>
<FontAwesomeIcon className="icon" icon={faLock} />
</button>
<button className ='btn' onClick={this.uncode}>
<FontAwesomeIcon className="icon" icon={faLockOpen} />
</button>
</div>
</div>
<div className="result">
{!this.state.changeText ? <textarea disabled className="textarea"></textarea> : <Cypher
text={this.state.text}
textCode={this.state.textCode}
/>}
</div>
</div>
</div>
);
}
}
export default Textarea;
<file_sep>/src/components/App.test.js
import React from 'react'
import { shallow } from 'enzyme'
import App from './App'
import { expect } from 'chai'
import sinon from 'sinon'
describe('Component App', () => {
it('should render Password', () => {
const wrapper = shallow(<App />)
expect(wrapper.find('Password'));
});
it('should render header', () => {
const wrapper = shallow(<App />)
expect(wrapper.find('header'));
});
});
<file_sep>/src/components/Textarea.test.js
import React from 'react'
import { shallow } from 'enzyme'
import Textarea from './TextArea'
import { expect } from 'chai'
import sinon from 'sinon'
describe('Component Textarea', () => {
it('should render Component Cypher', () => {
const wrapper = shallow(<Textarea />)
expect(wrapper.find('Cypher'));
});
it('should render button', () => {
const wrapper = shallow(<Textarea />)
expect(wrapper.find('button'));
});
});
<file_sep>/README.md
Dzień dobry wszystkim! :)
Każdy projekt uczy czegoś nowego, dziękuję synapsi.xyz za możliwość jego wykonania!
Hasło potrzebne do uzyskania autoryzacji i skorzystania z generatora szyfru to: !<EMAIL>
Miłego szyfrowania i odszyfrowywania :)
Zadanie zostało wykonane za pomocą React JS.
<NAME>
<file_sep>/src/components/Password.test.js
import React from 'react'
import { shallow } from 'enzyme'
import Password from './Password'
import { expect } from 'chai'
import sinon from 'sinon'
describe('Component Password', () => {
it('should render Textarea', () => {
const wrapper = shallow(<Password />)
expect(wrapper.find('Textarea'));
});
it('should render Authorization', () => {
const wrapper = shallow(<Password />)
expect(wrapper.find('h1').first().text()).to.contain('Authorization');
});
it('should render div which contains input', () => {
const wrapper = shallow(<Password />)
const input = wrapper.find('input')
expect(wrapper.find('div')).to.contain(input);
});
});
| 83f6b8ea460222fb1450eae89a368fbac043fd61 | [
"JavaScript",
"Markdown"
] | 6 | JavaScript | paweljankowski92/task-synapsi | 4e2920cc82ae9c5794c27d5f6716a930ed4fbbb8 | f3a34a1ef87e7c9159447d4719ac74d2fd94611f | |
refs/heads/master | <file_sep>import React, { Component } from 'react'
import {Navbar,Nav} from 'react-bootstrap';
import {NavLink} from 'react-router-dom';
import './Header.scss'
export default class Header extends Component {
render() {
return (
<Navbar bg="primary" variant="dark" className="">
<Navbar.Brand className="nav-brand">Navbar</Navbar.Brand>
<Nav className=" mr-auto ">
<Nav.Link >
<NavLink to="/dashboard" className="nav-link">Dashboard </NavLink>
</Nav.Link>
</Nav>
<Nav className="ml-auto left-content">
<Nav.Link >
<NavLink to="/login" className="nav-link">Login </NavLink>
</Nav.Link>
<Nav.Link >
<NavLink to="/signup" className="nav-link">SignUp</NavLink>
</Nav.Link>
</Nav>
</Navbar>
)
}
}
| ac80541b1e91ae4afb2bb31f91ae49eb860dbcb9 | [
"JavaScript"
] | 1 | JavaScript | gsrrinathreddy/kanbanboard-react-springboot | ceff07d2a0232a0dfc0c1e8dcf16745f6f054c1e | df936586c08020a356b8ccae41d8f068507f0d0d | |
refs/heads/master | <file_sep>//
// Consst.h
// MAX+
//
// Created by tarena on 16/8/10.
// Copyright © 2016年 tarena. All rights reserved.
//
#ifndef Consst_h
#define Consst_h
//屏幕 宽 高
#define kMainWindowWidth [UIScreen mainScreen].bounds.size.width
#define kMainWindowHeight [UIScreen mainScreen].bounds.size.height
//RGBA Color
#define krgb(r, g, b, a) [UIColor colorWithRed:r/255.0 green:g/255.0 blue:b/255.0 alpha:a]
#endif /* Consst_h */
<file_sep># Uncomment this line to define a global platform for your project
# platform :ios, '9.0'
target 'MAX+' do
# Uncomment this line if you're using Swift or would like to use dynamic frameworks
# use_frameworks!
# Pods for MAX+
pod 'AFNetworking'
pod 'MJRefresh'
pod 'Masonry'
pod 'BlocksKit'
pod 'CocoaLumberjack'
pod 'MBProgressHUD'
pod 'TPKeyboardAvoiding'
pod 'MLTransition'
pod 'YYKit'
pod 'iCarousel'
end
<file_sep>//
// RequestPath.h
// MAX+
//
// Created by tarena on 16/8/9.
// Copyright © 2016年 tarena. All rights reserved.
//
#ifndef RequestPath_h
#define RequestPath_h
#define kBasePath @"http://app3.qdaily.com"
#define kMainPath @"/app3/homes/index/%@.json?"
#define kOtherPath @"/app3/columns/index/%ld/%@.json?"
#define kimgBasePath @"http://cache.tuwan.com"
#define kimgPath @"/app/?appid=1&class=cos&mod=cos&classmore=indexpic&dtid=0&appid=1&appver=2.1&start=%ld"
#define kVideoPath @"http://live.9158.com/Room/GetHotLive_v2?type=1&page=%ld&lat=0.0&lon=0.0"
#endif /* RequestPath_h */
| 1b91570ca8f6d27fc129f6211f96376d9f789417 | [
"C",
"Ruby"
] | 3 | C | sChao001/Zahuopu | f5ace20df3236f5a5515322150bc005b296dd29c | b645b1daed1855e5bd10975aa75cd606711dc777 | |
refs/heads/master | <file_sep>from io import StringIO
__author__ = 'zz'
from email.parser import Parser
def parsestr(self, text, headersonly=False):
text = text.encode('latin1').decode()
return self.parse(StringIO(text), headersonly=headersonly)
def make_fix_encoding():
Parser.parsestr = parsestr
<file_sep>__author__ = 'zz'
from email.feedparser import BufferedSubFile
import re
from itertools import zip_longest
sep = re.compile(r'(\r\n|\r|\n)')
def py3_splitlines(s):
split_group = sep.split(s)
return [g1 + g2 for g1, g2 in zip_longest(split_group[::2], split_group[1::2], fillvalue='')]
# monkey patch the push method
def push(self, data):
"""Push some new data into this object."""
# Crack into lines, but preserve the linesep characters on the end of each
# parts = data.splitlines(True)
# use py3_splitlines instead of the str.splitlines
parts = py3_splitlines(data)
if not parts or not parts[0].endswith(('\n', '\r')):
# No new complete lines, so just accumulate partials
self._partial += parts
return
if self._partial:
# If there are previous leftovers, complete them now
self._partial.append(parts[0])
# and here
parts[0:1] = py3_splitlines(''.join(self._partial))
del self._partial[:]
# If the last element of the list does not end in a newline, then treat
# it as a partial line. We only check for '\n' here because a line
# ending with '\r' might be a line that was split in the middle of a
# '\r\n' sequence (see bugs 1555570 and 1721862).
if not parts[-1].endswith('\n'):
self._partial = [parts.pop()]
self.pushlines(parts)
def make_fix_splitlines():
BufferedSubFile.push = push
<file_sep>__author__ = 'zz'
from setuptools import setup
setup(
name='fix_headers_parse',
version=0.1,
author='zz',
author_email='<EMAIL>',
url='https://github.com/littlezz/fix-headers-parse',
description='monkey patch to fix python3 headers parse when headers contain some special character, eg, some chinese',
packages=['fix_headers_parse'],
license='MIT',
)
<file_sep>__author__ = 'zz'
_all_fix = ('fix_encoding', 'fix_splitlines')
def _redirect_name(name):
return 'make_' + name
def make_headers_fix(fix_names=None):
from .fix_encoding import make_fix_encoding
from .fix_splitlines import make_fix_splitlines
if fix_names is None:
fix_names = _all_fix
for name in fix_names:
locals()[_redirect_name(name)]()<file_sep>https://github.com/FrederickPullen/fix-headers-parse.git
<file_sep># fix-headers-parse
monkey patch to fix python3 headers parse when headers contain some special character , eg, some chinese
This module try to use monkey patch to replace the behave of `push` method in
the `email.feedparser.BufferedSubFile` to avoid headers split by `\x85` (fix_splitlines)
And use utf8 to decode the headers (fix_encoding)
Install
------------
You need pip to install this library.
```
pip3 install git+https://github.com/littlezz/fix-headers-parse
```
Quick Start
---------------
fix splitlines and encoding
```python
from fix_headers_parse import make_headers_fix
make_headers_fix()
```
or just explicit fix 'splitlines'
```python
make_headers_fix('fix_splitlines')
```
here is list of fix:
- fix_splitlines
- fix_encoding
Why this library
---------
python3 use latin-1 to decode the headers, if some Chinese encode by utf8, but decode by latin-1, it may contain `\x85` in the result.
```python
In [276]: '锅团子圣诞树.jpg'.encode('utf8').decode('latin1')
Out[276]: 'é\x94\x85å\x9b¢å\xad\x90å\x9c£è¯\x9eæ\xa0\x91.jpg'
In [278]: '\x85' in '锅团子圣诞树.jpg'.encode('utf8').decode('latin1')
Out[278]: True
```
In `email.feedparser.BufferedSubFile`, the push method split data by `str.splitlines` , which will split on `\x85`. (https://docs.python.org/3.5/library/stdtypes.html#str.splitlines)
This will make headers content lost after the `\x85`.
I write a simple server to return Chinese headers encode by utf8.
```python
from flask import Flask, make_response
app = Flask(__name__)
@app.route('/rt')
def rt():
r = make_response()
r.headers['chinese-header'] = '锅团子圣诞树.jpg'.encode('utf8')
return r
if __name__ == '__main__':
app.run(port=8088, debug=True)
```
and then get it.
```python
In [275]: requests.get('http://127.0.0.1:8088/rt').headers['chinese-header']
Out[275]: 'é\x94\x85'
```
It lost content after `\x85`.
I write a function to replace the `push` method, it replace the `str.splitlines` to split only on `\r`, `\n`, `\r\n`.
```python
__author__ = 'zz'
from email.feedparser import BufferedSubFile
import re
from itertools import zip_longest
sep = re.compile(r'(\r\n|\r|\n)')
def py3_splitlines(s):
split_group = sep.split(s)
return [g1 + g2 for g1, g2 in zip_longest(split_group[::2], split_group[1::2], fillvalue='')]
# monkey patch the push method
def push(self, data):
"""Push some new data into this object."""
# Crack into lines, but preserve the linesep characters on the end of each
# parts = data.splitlines(True)
# use py3_splitlines instead of the str.splitlines
parts = py3_splitlines(data)
if not parts or not parts[0].endswith(('\n', '\r')):
# No new complete lines, so just accumulate partials
self._partial += parts
return
if self._partial:
# If there are previous leftovers, complete them now
self._partial.append(parts[0])
# and here
parts[0:1] = py3_splitlines(''.join(self._partial))
del self._partial[:]
# If the last element of the list does not end in a newline, then treat
# it as a partial line. We only check for '\n' here because a line
# ending with '\r' might be a line that was split in the middle of a
# '\r\n' sequence (see bugs 1555570 and 1721862).
if not parts[-1].endswith('\n'):
self._partial = [parts.pop()]
self.pushlines(parts)
BufferedSubFile.push = push
```
after replace the `splitlines` to `py3_splitlines` in `push` method.
```python
In [280]: requests.get('http://127.0.0.1:8088/rt').headers['chinese-header']
Out[280]: 'é\x94\x85å\x9b¢å\xad\x90å\x9c£è¯\x9eæ\xa0\x91.jpg'
```
and then we can re-encode the headers and get the correct one
So, I think we can use monkey patch to fix the `BufferedSubFile.push` method when people use python3.
I also find that the str.splitlines in `BufferedSubFile.push` may be a bug (http://bugs.python.org/issue22233).
But until now, it seems that python source code doesn't change.
| 6fbfe759088bfdfedbf596aceae5c7450eb0c6e8 | [
"Markdown",
"Python"
] | 6 | Python | FrederickPullen/fix-headers-parse | 03f9b97f0888ab4692edaaac4be1bc3feb380102 | fdb022d38cb60d8a651202aa7983e97643ca77b9 | |
refs/heads/master | <file_sep>/*!
* Copyright 2016, nju33
* Released under the MIT License
* https://github.com/totora0155/maru.js
*/
!function(t,e){"object"==typeof exports&&"undefined"!=typeof module?module.exports=e():"function"==typeof define&&define.amd?define(e):t.Maru=e()}(this,function(){"use strict";
// var box;
function t(t){if(this.el=el,this.opts={timeout:200},this._cache={},this._event,t.timeout){if("number"==typeof t.timeout)throw Error("`timeout` must be an integer");this.opts.timeout=t.timeout}}function e(t){
// TODO: user customize
t.style.cssText="position:fixed;right:50%;bottom:50%;-webkit-transform:translate(50%,50%);transform:translate(50%,50%);transition: opaicty .2s linear"}
// function init() {
// box = document.createElement('div');
// box.className = 'maru__box';
// injectStyle();
// }
function i(t){t.style.opacity=1}function n(t){requestAnimationFrame?requestAnimationFrame(t):setTimeout(t,0)}var o={ID:"data-maru-id"};
// function injectStyle() {
// var style = document.createElement('style');
// var css = '';
// style.innerText = css;
// document.head.insertBefore(style, document.head.children[0]);
// }
return maru.prototype.regist=function(t){this._.event=t},maru.prototype.open=function(t){var r=t.currentTarget,s=r.getAttribute(o.ID),u=null;if(this._cache[s])u=this._cache[s];else{if(u=document.getElementById(s),!u)throw Error(u+" is undefined");e(u)}u.style.display="block",this._event&&"object"==typeof this._event&&Object.keys(this._event).forEach(function(t){try{var e=document.getElementById(t),i=this._event[t].action,n=this._event[t].handle;e.addEventListener(i,n,!1)}catch(o){throw Error(o)}}),n(function(){move(this.el),i(this.el)}.bind(this))},menu.prototype.close=function(){this._event&&"object"==typeof this._event&&Object.keys(this._event).forEach(function(t){try{var e=document.getElementById(t),i=this._event[t].action,n=this._event[t].handle;e.removeEventListener(i,n,!1)}catch(o){throw Error(o)}}),setTimeout(function(){this.el.style.display="none"}.bind(thid),this.opts.timeout)},t});<file_sep>const banner = `
/*!
* Copyright 2016, nju33
* Released under the MIT License
* https://github.com/totora0155/maru.js
*/
`;
export default {
banner: banner.trim(),
entry: 'lib/maru.js',
format: 'umd',
dest: 'dist/maru.js',
moduleName: 'Maru',
};
<file_sep># Maru.js
<file_sep>/*!
* Copyright 2016, nju33
* Released under the MIT License
* https://github.com/totora0155/maru.js
*/
(function (global, factory) {
typeof exports === 'object' && typeof module !== 'undefined' ? module.exports = factory() :
typeof define === 'function' && define.amd ? define(factory) :
(global.Maru = factory());
}(this, function () { 'use strict';
var customAttr = {
ID: 'data-maru-id',
}
// var box;
function Maru(opts) {
this.el = el;
this.opts = {
timeout: 200,
};
this._cache = {};
this._event;
if (opts.timeout) {
if (typeof opts.timeout === 'number') {
throw Error('`timeout` must be an integer')
}
this.opts.timeout = opts.timeout;
}
// if (!box) {
// init();
// }
};
maru.prototype.regist = function regist(event) {
this._.event = event;
}
maru.prototype.open = function open(e) {
var target = e.currentTarget;
var id = target.getAttribute(customAttr.ID);
var el = null;
if (this._cache[id]) {
el = this._cache[id];
} else {
el = document.getElementById(id);
if (!el) {
throw Error(el + ' is undefined');
}
init(el);
}
el.style.display = 'block';
if (this._event && typeof this._event === 'object') {
Object
.keys(this._event)
.forEach(function(id) {
try {
var el = document.getElementById(id)
var action = this._event[id].action;
var handle = this._event[id].handle;
el.addEventListener(action, handle, false);
} catch (e) {
throw Error(e)
}
});
}
next(function () {
move(this.el);
show(this.el);
}.bind(this));
};
menu.prototype.close = function () {
if (this._event && typeof this._event === 'object') {
Object
.keys(this._event)
.forEach(function(id) {
try {
var el = document.getElementById(id)
var action = this._event[id].action;
var handle = this._event[id].handle;
el.removeEventListener(action, handle, false);
} catch (e) {
throw Error(e)
}
});
}
setTimeout(function() {
this.el.style.display = 'none';
}.bind(thid), this.opts.timeout);
}
function init(el) {
// TODO: user customize
el.style.cssText = 'position:fixed;' +
'right:50%;' +
'bottom:50%;' +
'-webkit-transform:translate(50%,50%);' +
'transform:translate(50%,50%);' +
'transition: opaicty .2s linear'
;
}
// function init() {
// box = document.createElement('div');
// box.className = 'maru__box';
// injectStyle();
// }
function show(el) {
el.style.opacity = 1;
// TODO:
}
function next(cb) {
if (requestAnimationFrame) {
requestAnimationFrame(cb);
} else {
setTimeout(cb, 0);
}
}
// function injectStyle() {
// var style = document.createElement('style');
// var css = '';
// style.innerText = css;
// document.head.insertBefore(style, document.head.children[0]);
// }
return Maru;
})); | cb2b1929c31b57ccafa40820b26f5380900540e2 | [
"JavaScript",
"Markdown"
] | 4 | JavaScript | totora0155/maru.js | a72e8f8a78733692119c82dfb2e228d8ca2b19dd | 3e6f2953d72d4e716650c95d8760d21d38528c11 | |
refs/heads/master | <file_sep>from django.db import models
class cinema(models.Model):
nama = models.CharField('Nama', max_length=27)
alamat = models.CharField('Alamat', max_length=100)
kontak = models.IntegerField()
def __str__(self):
return self.nama
def __iter__(self):
return iter([self.nama,
self.alamat,
self.kontak
])
class movie(models.Model):
judul = models.CharField('Judul', max_length=100)
kategori = models.CharField('Kategori', max_length=100)
tahun = models.IntegerField()
def __str__(self):
return self.judul
def __iter__(self):
return iter([self.judul,
self.kategori,
self.tahun
])
class showing(models.Model):
showing_time = models.TimeField()
showing_date = models.DateField()
movies = models.ForeignKey(movie, on_delete=models.CASCADE)
def __str__(self):
return self.movies.judul
class theater(models.Model):
nama = models.CharField('Nama', max_length=27)
kapasitas = models.IntegerField()
cinemas = models.ForeignKey(cinema, on_delete=models.CASCADE)
showings = models.ManyToManyField(showing)
def __str__(self):
return self.nama
class ticket(models.Model):
nomor_kursi = models.CharField(max_length=10)
harga = models.IntegerField()
showings = models.ForeignKey(showing, on_delete=models.CASCADE)
def __str__(self):
return self.nomor_kursi<file_sep>"""Kota URL Configuration
The `urlpatterns` list routes URLs to views. For more information please see:
https://docs.djangoproject.com/en/1.11/topics/http/urls/
Examples:
Function views
1. Add an import: from my_app import views
2. Add a URL to urlpatterns: url(r'^$', views.home, name='home')
Class-based views
1. Add an import: from other_app.views import Home
2. Add a URL to urlpatterns: url(r'^$', Home.as_view(), name='home')
Including another URLconf
1. Import the include() function: from django.conf.urls import url, include
2. Add a URL to urlpatterns: url(r'^blog/', include('blog.urls'))
"""
from django.conf.urls import url, include
from django.contrib import admin
from MovieTheater import views
urlpatterns = [
url(r'^admin/', admin.site.urls),
url(r'^api/cinema/add/$', views.add_cinema),
url(r'^api/showing/add/$', views.add_showing),
url(r'^api/movie/add/$', views.add_movie),
url(r'^api/cinema/$', views.get_all_cinema),
url(r'^api/cinema/(?P<id>[^/]*)/$', views.get_cinema),
url(r'^api/movie/$', views.get_all_movie),
url(r'^api/movie/(?P<id>[^/]*)/$', views.get_movie),
url(r'^api/showing/$', views.get_all_showing),
url(r'^api/showing/(?P<id>[^/]*)/$', views.get_showing),
url(r'^api/theater/$', views.get_all_theater),
url(r'^api/theater/(?P<id>[^/]*)/$', views.get_theater),
url(r'^api/ticket/$', views.get_all_ticket),
url(r'^api/ticket/(?P<id>[^/]*)/$', views.get_ticket),
]
<file_sep>from django.contrib import admin
from . models import *
admin.site.register(cinema)
admin.site.register(movie)
admin.site.register(showing)
admin.site.register(theater)
admin.site.register(ticket)<file_sep>from django.shortcuts import render
from MovieTheater.serializers import *
from rest_framework.response import Response
from rest_framework.decorators import api_view
from .models import *
from rest_framework import status
@api_view(['GET'])
def get_all_cinema(request):
cinemas = cinema.objects.all()
serializer = cinemaSerializer(cinemas, many= True).data
return Response({"data" : serializer}, status=status.HTTP_200_OK)
@api_view(['GET', 'PUT', 'DELETE'])
def get_cinema(request, id):
try:
cinema1 = cinema.objects.get(id=id)
except cinema.DoesNotExist:
return Response(status=status.HTTP_404_NOT_FOUND)
if request.method == 'GET':
serial = cinemaSerializer(cinema1).data
return Response({"data" : serial}, status=status.HTTP_200_OK)
elif request.method == 'PUT':
serializer = cinemaSerializer(cinema1, data=request.data)
if serializer.is_valid():
serializer.save()
return Response({"data" : serializer.data}, status=status.HTTP_200_OK)
return Response(serializer.errors, status=status.HTTP_400_BAD_REQUEST)
elif request.method == 'DELETE':
cinema1.delete()
return Response(status=status.HTTP_204_NO_CONTENT)
@api_view(['GET'])
def get_all_movie(request):
movies = movie.objects.all()
serializer = movieSerializer(movies, many= True).data
return Response({"data" : serializer}, status=status.HTTP_200_OK)
@api_view(['GET', 'PUT', 'DELETE'])
def get_movie(request, id):
try:
movie1 = movie.objects.get(id=id)
except movie.DoesNotExist:
return Response(status=status.HTTP_404_NOT_FOUND)
if request.method == 'GET':
serializer = movieSerializer(movie1).data
return Response({"data" : serializer}, status=status.HTTP_200_OK)
elif request.method == 'PUT':
serializer = movieSerializer(movie1, data=request.data)
if serializer.is_valid():
serializer.save()
return Response({"data" : serializer.data}, status=status.HTTP_200_OK)
return Response(serializer.errors, status=status.HTTP_400_BAD_REQUEST)
elif request.method == 'DELETE':
movie1.delete()
return Response(status=status.HTTP_204_NO_CONTENT)
@api_view(['GET'])
def get_all_showing(request):
showings = showing.objects.all()
serializer = showingSerializer(showings, many= True).data
return Response({"data" : serializer}, status=status.HTTP_200_OK)
@api_view(['GET', 'PUT', 'DELETE'])
def get_showing(request, id):
try:
showing1 = showing.objects.get(id=id)
except showing.DoesNotExist:
return Response(status=status.HTTP_404_NOT_FOUND)
if request.method == 'GET':
serializer = showingSerializer(showing1).data
return Response({"data" : serializer}, status=status.HTTP_200_OK)
elif request.method == 'PUT':
serializer = showingSerializer(showing1, data=request.data)
if serializer.is_valid():
serializer.save()
return Response({"data" : serializer.data}, status=status.HTTP_200_OK)
return Response(serializer.errors, status=status.HTTP_400_BAD_REQUEST)
elif request.method == 'DELETE':
showing1.delete()
return Response(status=status.HTTP_204_NO_CONTENT)
@api_view(['POST'])
def add_cinema(request):
cinema = cinemaSerializer(data = request.data)
if cinema.is_valid():
cinema.save()
return Response({"data" : "Berhasil Memasukkan Cinema"}, status=status.HTTP_201_CREATED)
else:
error_details = []
for key in cinema.errors.keys():
error_details.append({"field": key, "message": cinema.errors[key][0]})
data = {
"Error": {
"status": 400,
"message": "Data tidak valid",
"error_details": error_details
}
}
return Response(data, status=status.HTTP_400_BAD_REQUEST)
@api_view(['POST'])
def add_movie(request):
movie = movieSerializer(data = request.data)
if movie.is_valid():
movie.save()
return Response({"data" : "Berhasil Memasukkan Movie"}, status=status.HTTP_201_CREATED)
else:
error_details = []
for key in movie.errors.keys():
error_details.append({"field": key, "message": movie.errors[key][0]})
data = {
"Error": {
"status": 400,
"message": "Data tidak valid",
"error_details": error_details
}
}
return Response(data, status=status.HTTP_400_BAD_REQUEST)
@api_view(['POST'])
def add_showing(request):
showing = showingSerializer(data = request.data)
if showing.is_valid():
showing.save()
return Response({"data" : "Berhasil Memasukkan Showing"}, status=status.HTTP_201_CREATED)
else:
error_details = []
for key in showing.errors.keys():
error_details.append({"field": key, "message": showing.errors[key][0]})
data = {
"Error": {
"status": 400,
"message": "Data tidak valid",
"error_details": error_details
}
}
return Response(data, status=status.HTTP_400_BAD_REQUEST)
@api_view(['GET'])
def get_all_theater(request):
theaters = theater.objects.all()
serializer = theaterSerializer(theaters, many= True).data
return Response({"data" : serializer}, status=status.HTTP_200_OK)
@api_view(['GET'])
def get_all_ticket(request):
tickets = ticket.objects.all()
serializer = ticketSerializer(tickets, many= True).data
return Response({"data" : serializer}, status=status.HTTP_200_OK)
@api_view(['GET', 'PUT', 'DELETE'])
def get_theater(request, id):
try:
theater1 = theater.objects.get(id=id)
except theater.DoesNotExist:
return Response(status=status.HTTP_404_NOT_FOUND)
if request.method == 'GET':
serializer = theaterSerializer(theater1).data
return Response({"data" : serializer}, status=status.HTTP_200_OK)
elif request.method == 'PUT':
serializer = theaterSerializer(theater1, data=request.data)
if serializer.is_valid():
serializer.save()
return Response({"data" : serializer.data}, status=status.HTTP_200_OK)
return Response(serializer.errors, status=status.HTTP_400_BAD_REQUEST)
elif request.method == 'DELETE':
theater1.delete()
return Response(status=status.HTTP_204_NO_CONTENT)
@api_view(['GET', 'PUT', 'DELETE'])
def get_ticket(request, id):
try:
ticket1 = ticket.objects.get(id=id)
except ticket.DoesNotExist:
return Response(status=status.HTTP_404_NOT_FOUND)
if request.method == 'GET':
serializer = ticketSerializer(ticket1).data
return Response({"data" : serializer}, status=status.HTTP_200_OK)
elif request.method == 'PUT':
serializer = ticketSerializer(ticket1, data=request.data)
if serializer.is_valid():
serializer.save()
return Response({"data" : serializer.data}, status=status.HTTP_200_OK)
return Response(serializer.errors, status=status.HTTP_400_BAD_REQUEST)
elif request.method == 'DELETE':
ticket1.delete()
return Response(status=status.HTTP_204_NO_CONTENT)<file_sep># -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2019-04-12 09:48
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
initial = True
dependencies = [
]
operations = [
migrations.CreateModel(
name='cinema',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('nama', models.CharField(max_length=27, verbose_name='Nama')),
('alamat', models.CharField(max_length=100, verbose_name='Alamat')),
('kontak', models.IntegerField()),
],
),
migrations.CreateModel(
name='movie',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('judul', models.CharField(max_length=100, verbose_name='Judul')),
('kategori', models.CharField(max_length=100, verbose_name='Kategori')),
('tahun', models.IntegerField()),
],
),
migrations.CreateModel(
name='showing',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('showing_time', models.TimeField()),
('showing_date', models.DateField()),
('movies', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='MovieTheater.movie')),
],
),
migrations.CreateModel(
name='theater',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('nama', models.CharField(max_length=27, verbose_name='Nama')),
('kapasitas', models.IntegerField()),
('cinemas', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='MovieTheater.cinema')),
('showings', models.ManyToManyField(to='MovieTheater.showing')),
],
),
migrations.CreateModel(
name='ticket',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('nomor_kursi', models.CharField(max_length=10)),
('harga', models.IntegerField()),
('showings', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='MovieTheater.showing')),
],
),
]
<file_sep>from rest_framework import serializers
from .models import *
class cinemaSerializer(serializers.ModelSerializer):
# nama = serializers.CharField(max_length=27)
# alamat = serializers.CharField(max_length=100)
# kontak = serializers.IntegerField()
class Meta:
model = cinema
fields = ('nama', 'alamat', 'kontak')
def create(self, validated_data):
cinemas = cinema(**validated_data)
cinemas.save()
return cinemas
class movieSerializer(serializers.ModelSerializer):
# judul = serializers.CharField(max_length=100)
# kategori = serializers.CharField( max_length=100)
# tahun = serializers.IntegerField()
class Meta:
model = movie
fields = '__all__'
def create(self, validated_data):
movies = movie(**validated_data)
movies.save()
return movies
class showingSerializer(serializers.ModelSerializer):
# showing_time = serializers.TimeField()
# showing_date = serializers.DateField()
# movies = serializers.ForeignKey(movie, on_delete=models.CASCADE)
movies = movieSerializer(many=False)
class Meta:
model = showing
fields = ('showing_time', 'showing_date', 'movies')
# fields = '__all__'
def create(self, validated_data):
movie_data = validated_data.pop('movies')
movie1 = movie.objects.get(**movie_data)
showings = showing.objects.create(movies=movie1, **validated_data)
return showings
# showings = showing(**validated_data)
# showings.save()
# return showings
def update(self, instance, validated_data):
instance.showing_time = validated_data.get('showing_time', instance.showing_time)
instance.showing_date = validated_data.get('showing_date', instance.showing_date)
movie_data = validated_data.pop('movies')
movie1 = movie.objects.get(**movie_data)
instance.movies = movie1
instance.save()
return instance
class theaterSerializer(serializers.ModelSerializer):
# nama = serializers.CharField(max_length=27)
# kapasitas = serializers.IntegerField()
# cinemas = serializers.ForeignKey(cinema, on_delete=models.CASCADE)
# showings = serializers.ManyToManyField(showing)
cinemas = cinemaSerializer(many=False, read_only=True)
showings = showingSerializer(many=True)
class Meta:
model = theater
fields = ('nama', 'kapasitas', 'cinemas', 'showings')
class ticketSerializer(serializers.ModelSerializer):
# nomor_kursi = serializers.CharField(max_length=10)
# harga = serializers.IntegerField()
# showings = serializers.ForeignKey(showing, on_delete=models.CASCADE)
showings = showingSerializer(many=False)
class Meta:
model = ticket
fields = '__all__'<file_sep>from django.apps import AppConfig
class MovietheaterConfig(AppConfig):
name = 'MovieTheater'
| 4eec6e1c44e37da4c6e0704094ee3345920b30e5 | [
"Python"
] | 7 | Python | purwoajifa/movieweb | 91f759570c2b4684b44510d20804c7f7ff8493b1 | eb8c0fcd179aec47cbf8171f1375885291d09a43 | |
refs/heads/master | <repo_name>markusbkoch/balancer-apy<file_sep>/bal_apy.py
import pandas as pd
import requests
import json
from web3 import Web3
import os
import sys
THEGRAPH_URL = 'https://api.thegraph.com/subgraphs/name/balancer-labs/balancer'
try:
web3_provider = os.environ['ENDPOINT_URL']
w3 = Web3(Web3.WebsocketProvider(web3_provider))
except:
sys.exit('You must set the ENDPOINT_URL environment variable')
def query_thegraph(query):
r = requests.post(THEGRAPH_URL, json = {'query':query})
try:
return json.loads(r.content)['data']
except:
print(query)
print(r.content)
raise
def get_pool_data(pools, block=0):
if type(pools) == str:
pools = [pools.lower()]
else:
pools = list(map(lambda x: x.lower(), pools))
if block==0:
query = '''
query {{
pools(first: 1000,
where: {{id_in: ["{0}"]}}) {{
id
liquidity
totalSwapFee
totalShares
}}
}}'''.format('","'.join(pools))
else:
query = '''
query {{
pools(first: 1000, block: {{number: {1}}},
where: {{id_in: ["{0}"]}}) {{
id
liquidity
totalSwapFee
totalShares
}}
}}'''.format('","'.join(pools), block)
p = query_thegraph(query)['pools']
df = pd.DataFrame(p).set_index('id').astype(float)
return df
def get_pools_APY(pools, end_block=None):
if end_block:
m = end_block
else:
m = w3.eth.getBlock('latest').number - 10 #can't use the actual latest because Subgraph lags behind
n = m-6600
t_m = w3.eth.getBlock(m).timestamp
t_n = w3.eth.getBlock(n).timestamp
m_data = get_pool_data(pools, m)#.set_index('id')
n_data = get_pool_data(pools, n)#.set_index('id')
merge = n_data.join(m_data, lsuffix='_n', rsuffix='_m')
merge.drop(columns=['liquidity_n','totalShares_n'], inplace=True)
merge['APY'] = 31556926 / (t_m - t_n) * (merge['totalSwapFee_m'] - merge['totalSwapFee_n']) / merge['liquidity_m']
return merge[['liquidity_m','totalShares_m','APY']]
def get_pools(liquidity_provider, block=0):
liquidity_provider = liquidity_provider.lower()
if block==0:
query = '''
query {{
users(first: 1000,
where: {{id: "{0}"}}) {{
sharesOwned (where: {{balance_gt: 0}}) {{
poolId {{id}}
balance
}}
}}
}}'''.format(liquidity_provider)
else:
query = '''
query {{
users(first: 1000, block: {{number: {1}}},
where: {{id: "{0}"}}) {{
sharesOwned (where: {{balance_gt: 0}}) {{
poolId {{id}}
balance
}}
}}
}}'''.format(liquidity_provider, block)
balances = query_thegraph(query)['users'][0]['sharesOwned']
balances = list(map(lambda x: (x['poolId']['id'], x['balance']), balances))
df = pd.DataFrame(balances)
df.columns = ['id', 'balance']
df = df.set_index('id').astype(float)
return df
def get_lp_APY(lp, block=0):
if block==0:
m = w3.eth.getBlock('latest').number - 10
else:
m = block
lp_pools = get_pools(lp, m)
lp_pools_apy = get_pools_APY(lp_pools.index.values, m)
merge = lp_pools_apy.join(lp_pools)
merge['lp_liquidity'] = merge['liquidity_m'] * merge['balance'] / merge['totalShares_m']
lp_apy = (merge['lp_liquidity'] * merge['APY']).sum() / merge['lp_liquidity'].sum()
return lp_apy
if sys.argv[1] == 'pool':
print(f'APY for pool {sys.argv[2]}')
a = get_pools_APY(sys.argv[2]).loc[sys.argv[2],'APY']
print('{:.2%}'.format(a))
if sys.argv[1] == 'lp':
print(f'APY for LP {sys.argv[2]}')
a = get_lp_APY(sys.argv[2])
print('{:.2%}'.format(a))
<file_sep>/explainer.md
# Computing APY
## Pool APY
### Approach
* compute the pool's revenue over the past 24h and assume it remains constant for the next 365 days;
* assume liquidity remains constant for the next 365 days;
* APY is the ratio between the 365 days expected revenue and the liquidity
### Formulas and data sources
$A_m$: Expected future APY at block $m$
$F_m$: `totalSwapFee` at block $m$
$F_n$: `totalSwapFee` at block $n$
$L_m$: `liquidity` at block $m$
$L_n$: `liquidity` at block $n$
$t_m$: `timestamp` of block $m$
$t_n$: `timestamp` of block $n$
1. Read $m$ from web3
2. Define $n=m-6500$ (roughly 24h worth of blocks)
3. Read $t_m$ and $t_n$ from web3
$$ A_m = \frac{31556926}{t_m - t_n}\cdot\frac{F_m-F_n}{L_m} $$
Alternatively, we could explore the pros and cons of an estimator of the average liquidity over the , eg. the average between the snapshot blocks
$$ A_m = \frac{31556926}{t_m - t_n}\cdot\ \frac{F_m-F_n}{\frac{L_m+L_n}{2}} $$
## Liquidity Provider's APY
### Approach
* compute the pool's APY for each pool the LP has liquidity in
* compute the share of the pool held by the LP
* The LP's APY is the weighted arithmetic mean of the APY of the pools they provided liquidity in (weights are the LP's liquidity in each pool)
### Formulas and data sources
$A_{l,m}$: Expected future APY of LP's $l$ position at block $m$
$A_{p,m}$: Expected future APY of pool $p$ at block $m$
$L_{p,m}$: `liquidity` of pool $p$ at block $m$
$L_{l,p,m}$: liquidity provided by LP $l$ at pool $p$ at block $m$
$S_{p,m}$: `totalShares` of pool $p$ at block $m$
$B_{l,p,m}$: `balance` of `PoolShare` for LP $l$ at pool $p$ at block $m$
1. Given an LP $X$, find the set of pools $P$ such that $B_{X,p,m}>0$ (query subgraph `users`)
2. For all pools in $P$, compute $L_{X,p,m}$ and $A_{p,m}$
$$ L_{X,p,m} = \frac{B_{X,p,m}L_{p,m}}{S_{p,m}} \forall{p\in{P}}$$
$$ A_{p,m} = \frac{31556926}{t_m - t_n}\cdot\frac{F_{p,m}-F_{p,n}}{L_{p,m}} \forall{p\in{P}}$$
3. Compute $A_{X,m}$
$$ A_{X,m} = \frac{\sum\limits_{p\in{P}}{A_{p,m}L_{X,p,m}}}{\sum{L_{X,p,m}}} $$<file_sep>/README.md
# Balancer APY
Simple script to compute APY of a pool or a liquidity provider's portfolio.
## Requirements
* Python 3
* An ethereum node (for querying blocks timestamps)
## Setup
* Install required packages: `pip install -r requirements.txt`
* Configure environment variable:
* `ENDPOINT_URL`: URL to an ethereum node that can be queried via Websockets
## Usage
### Pool APY
`python3 bal_apy.py pool <pool_address>`
### Liquidity Provider's APY
`python3 bal_apy.py lp <liquidity_provider_address>` | 231b7d409c85e80c73eec75f5afd6c75f99d8545 | [
"Markdown",
"Python"
] | 3 | Python | markusbkoch/balancer-apy | 0c51d25c3e74585d69c12d510a33cdd858a5811d | 122c99fa4c2644961b265606454414d95f287bc4 | |
refs/heads/master | <repo_name>2422002753/8.16<file_sep>/src/user1/mutations-types.js
// user1/mutations-types.js
// 把mutations事件名定义为常量 防止项目过大的时候大家的命名冲突
// eslint-disable-next-line import/prefer-default-export
export const MADD = 'MADD';
// 点击加的事件
<file_sep>/src/api/index.js
// api/index.js
// eslint-disable-next-line import/no-cycle
import myAjax from '../lib/axios';
const getList = () => myAjax.request({
url: '/list',
});
// 请求路由权限
const getAuth = () => myAjax.request({
url: '/auth',
});
// eslint-disable-next-line import/prefer-default-export
export { getList, getAuth };
<file_sep>/路由权限.md
component 新建 Icon.vue
# vue 路由权限
1. 后端返回权限列表
```js
[
{
parentId: -1,
name: '购物车',
id: 1,
auth: 'cart' //auth权限的标志,为了防范auth对应的都是路由path
},
{
parentId: 1,
id: 2,
name: '购物车列表',
auth: 'cart-list'
},
{
parentId: -1,
name: '我的',
id: 3,
auth: 'my' //auth权限的标志
}
];
```
2. 前端配置好路由
配置公共路由和权限路由
代码见:router.js
3. 获取权限
- stroe 定义一个值 这个值是代表有没有获取过权限如果没有就请求权限接口
- 请求接口
- 对比权限路由和权限列表来筛选出我们需要的路由
4. 通过 router.addRoutes 添加动态路由 也就是我们筛选出来的路由列表
<file_sep>/权限管理的副本.MD
Vue 权限菜单及按钮权限
# 一.服务端返回路由权限数据
Vue 权限菜单需要根据后端返回的数据来实现
[
{pid:-1,name:'购物车',id:1,auth:'cart'},
{pid:1,name:'购物车列表',id:4,auth:'cart-list'},
{pid:4,name:'彩票',id:5,auth:'lottery'},
{pid:4,name:'商品',id:6,auth:'product'},
{pid:-1,name:'商店',id:2,auth:'shop'},
{pid:-1,name:'个人中心',id:3,auth:'store'},
];
# 二.静态菜单
使用 element-ui 构建静态菜单
import ElementUI from 'element-ui';
import 'element-ui/lib/theme-chalk/index.css';
Vue.use(ElementUI);
<el-menu default-active="2" class="el-menu-vertical-demo">
<el-submenu index="1">
<template slot="title">导航一</template>
<el-submenu index="1-1">
<template slot="title">选项 1-1</template>
<el-menu-item index="1-1-1">选项 1-1-1</el-menu-item>
<el-menu-item index="1-1-2">选项 1-1-2</el-menu-item>
</el-submenu>
<el-menu-item index="1-2">选项 1-2</el-menu-item>
</el-submenu>
<el-menu-item index="2">
导航二
</el-menu-item>
<el-menu-item index="3">
导航三
</el-menu-item>
<el-menu-item index="4">
导航四
</el-menu-item>
</el-menu>
路由配置
import Vue from 'vue'
import Router from 'vue-router'
import Home from './views/Home.vue'
Vue.use(Router)
export const authRoutes = [ // 权限路由
{
path: '/cart',
name: 'cart',
component: () => import('@/views/Cart'),
children: [
{
path: 'cart-list',
name: 'cart-list',
component: () => import('@/views/CartList'),
children: [
{
path: 'lottery',
name: 'lottery',
component: () => import('@/views/Lottery'),
},
{
path: 'product',
name: 'product',
component: () => import('@/views/Product'),
},
],
},
],
},
];
export default new Router({ // 默认导出 首页和 404 页面
mode: 'history',
base: process.env.BASE_URL,
routes: [
{
path: '/',
name: 'home',
component: Home
},
{
path:'*',
component:{
render:h=>h('h1',{},'Not Found')
}
}
]
})
# 三.获取权限
根据后端返回的数据,格式化树结构,并提取用户权限
// 默认设置没有获取过权限
export default new Vuex.Store({
state: {
hasPermission:false
},
mutations: {
setPermission(state){
state.hasPermission = true
}
},
})
在路由跳转前看下是否获取过权限,如果没有获取过,就获取权限存入 vuex 中
router.beforeEach(async (to,from,next)=>{
if(!store.state.hasPermission){
// 获取最新路由列表
let newRoutes = await store.dispatch('getRouteList');
router.addRoutes(newRoutes); // 增加新路由
next({...to,replace:true})
}else{
next(); // 获取过就不需要再次获取了
}
})
# 四.获取相关需要数据
const getMenListAndAuth = (menuList)=>{
let menu = [];
let sourceMap = {};
let auth = [];
menuList.forEach(m => {
m.children = []; // 增加孩子列表
sourceMap[m.id] = m;
auth.push(m.auth)
if(m.pid === -1){
menu.push(m); // 根节点
}else{
sourceMap[m.pid] && sourceMap[m.pid].children.push(m)
}
});
return {menu,auth} // 获取菜单数据和权限数据
}
async getRouteList({dispatch,commit}){
let auths = await axios.get('http://localhost:3000/roleAuth');
let menuList = auths.data.menuList;
let {menu,auth} = getMenListAndAuth(menuList);
}
# 五.找到需要添加的路由
import {authRoutes} from './router'
const getRoutes = auth => {
const filter = (authRoutes)=>{
return authRoutes.filter(route=>{
// 包含权限
if(auth.includes(route.name)){
if(route.children){
route.children = filter(route.children);
}
return true;
}
})
}
return filter(authRoutes);
};
// 获取需要添加的路由列表
async getRouteList({ dispatch, commit }) {
let auths = await axios.get("http://localhost:3000/roleAuth");
let menuList = auths.data.menuList;
let { menu, auth } = getMenListAndAuth(menuList);
commit("setMenu", menu); // 将菜单数据保存起来
commit("setPermission"); // 权限获取完毕
// 通过 auth 查找需要添加的路由
return getRoutes(auth);
}
# 六.递归渲染菜单
渲染 Menu 组件提取公共部分
<template>
<div>
<el-menu>
<template v-for="menu in $store.state.menu">
<el-submenu v-if="menu.children.length" :key="menu.auth" :index="menu.auth">
<template slot="title">{{menu.name}}</template>
<!-- 此处需要不停的递归 el-submenu -->
</el-submenu>
<el-menu-item v-else :key="menu.auth" :index="menu.auth">{{menu.name}}</el-menu-item>
</template>
</el-menu>
</div>
</template>
编写递归组件
<template>
<el-submenu :index="menu.auth">
<template slot="title">{{menu.name}}</template>
<template v-for="(child,index) in menu.children">
<el-menu-item v-if="!child.children.length" :key="index">
<router-link :to="{name:child.auth}"> {{child.name}}</router-link>
</el-menu-item>
<!-- 如果有儿子继续递归组件 -->
<ResubMenu :menu="child" v-else :key="index"></ResubMenu>
</template>
</el-submenu>
</template>
<script>
export default {
name:'ResubMenu',
props:{
menu:{}
}
}
</script>
# 七.权限按钮控制
state: {
hasPermission: false,
menu: [], // 菜单权限
btnPermission:{ // 按钮权限
edit:false,
add:true
}
},
查看当前按钮是否有权限
<el-button v-has="'edit'">编辑</el-button>
<el-button v-has="'add'">添加</el-button>
自定义指令的使用
directives: {
has: {
inserted(el, bindings, vnode) {
let value = bindings.value;
// 在 vuex 中查看是否有按钮权限
let flag = vnode.context.\$store.state.btnPermission[value];
// 如果没有全选则将按钮删除即可
!flag && el.parentNode.removeChild(el);
}
}
}
<file_sep>/deploy.sh
#!/usr/bin/env sh
# 确保脚本抛出遇到的错误
set -e
echo '开始'
git add -A
echo '添加完成'
git commit -m 'vuex模块2拆分'
echo 'commit 完成'
git push origin master
echo 'push完成'
<file_sep>/src/mock/home.js
// mock/home.js 只处理首页的请求
// request 请求 response 响应
// url: "/list?id=1&name=lili" {id:1,name:'lili'}
import Mock from 'mockjs';
// 返回路由权限数组
const data = Mock.mock({
'list|3': [
{
name: '@cname',
email: '@email',
'age|10-20': 1,
},
],
});
function senList() {
return {
code: 200,
data,
};
}
function loginApi(req) {
const { user, pass } = JSON.parse(req.body);
// 判断用户名和密码是否合法
if (user === 'lili' && pass === '<PASSWORD>') {
return { msg: '登录成功' };
}
return { msg: '登录失败' };
}
export { senList, loginApi };
<file_sep>/vue组件传值.md
# vue 组件传值
1. props 父传子 数字、对象、数组、布尔值、对象的属性 都需要 v-bind: 缩写成 :
2. $emit 和 $on 子传父
```js
//子组件 更新m的值到200 .sync是一种语法糖
this.$emit('update:m', 200);
//父组件 money 和子组件的m值同步
<Son :m.sync="money"></Son>
```
```js
//子组件 v-model 只能针对value
this.$emit('input', 200);
// 父组件通过@value @input的事件进行修改
<Son @value='money'
@input="change"></Son>
// 事件
change(value) {
this.money = value;
}
//可以简写成下面
<Son v-model='money'></Son>
```
3. eventbus 简单项目可以用 eventbus 进行传值
- 把\$bus 变成一个 vue 的实例 同一个 vue 实例
```js
Vue.prototype.$bus = new Vue();
this.$bus.$emit('toS');
this.$bus.$on('toS', () => {
console.log(345);
});
```
4. refs 组件和 dom 上
ref 如果放在组件上 拿到的是挂到的组件上的实例
ref 如果放在元素上 拿到是当前元素的 dom
5. $attrs 和 $listeners 从上到下传递
$attrs 传递属性 继续往下传 用 v-bind
$listeners 传递的是事件 继续往下传 v-on
6. slot
7. vuex 所有组件都能共享状态
//作业 辅助函数的的模块使用 mapActions mapMumations MapGetters
rootState 和 rootGetters 可以拿到根 vuex 的 state 和 getters
// commit 派发到全局 作业
<file_sep>/README.md
# axios 封装
axios.create() 会创建一个 axios 实例
# mockjs 的使用
1. src 文件目录下面建立 mock 文件夹,mock 下面创建 index.js 文件
2. main.js 引入 mock/index.js
```js
//根据环境变量决定是否使用mock进行数据模拟
if(process.env.NODE_ENV=='development')import('./mock')
import() //es6 里面 懒加载
```
3. src 里面建立一个 api 文件夹 文件夹里面创建 index.js (放请求)
4. mockjs 可以拦截 ajax 请求 可以拦截匹配到的路径 可以一个页面 拆分出来一个 js 去做响应处理/或者一个功能模块拆分成一个 js
5. src 建立一个 lib 文件夹 工具类
作业 博客 写 axios 封装过程 vuex 使用
<file_sep>/src/mock/routerauth.js
// routerautu.js
// pid -1 表示一级路由 pid的父请等于上级的id auth 有权限就会有auth 标志
const authlist = () => [
{
parentid: -1,
name: '购物车',
id: 1,
auth: 'cart',
},
{
parentid: 1,
name: '购物车列表',
id: 4,
auth: 'cart-list',
},
{
parentid: 4,
name: '彩票',
id: 5,
auth: 'lott',
},
{
parentid: 4,
name: '商品',
id: 6,
auth: 'product',
},
{
parentid: -1,
name: 'my',
id: 2,
auth: 'cart',
},
];
export { authlist };
export default {};
<file_sep>/src/lib/util.js
const util = {
/**
* @param {*} str type:String
*/
queryString(str) {
if (typeof str !== 'string') {
throw TypeError('str is not String');
}
// eslint-disable-next-line no-param-reassign
str = str.split('?')[1].split('&');
const obj = {};
str.forEach((item) => {
// eslint-disable-next-line no-param-reassign
item = item.split('=');
// eslint-disable-next-line prefer-destructuring
obj[item[0]] = item[1];
});
return obj;
},
};
export default util;
<file_sep>/src/api/a.js
const router = [
{
name: 'cart',
children: [
{
name: 'list',
},
{
name: 'a',
},
],
},
{
name: 'ccc',
},
];
const auth = ['list', 'a'];
function format(router) {
return router.filter((item) => {
if (auth.includes(item.name)) {
// 过滤儿子
if (item.children) {
item.children = format(item.children);
}
return true;
}
});
}
const res = format(router);
console.log(res);
<file_sep>/src/mock/router.js
// routerautu.js
const authlist = () => [
// pid -1 表示一级路由 pid的父请等于上级的id auth 有权限就会有auth
{
pid: -1,
name: '购物车',
id: 1,
auth: 'cart',
},
{
pid: 1,
name: '购物车列表',
id: 4,
auth: 'cart-list',
},
{
pid: 4,
name: '彩票',
id: 5,
auth: 'lott',
},
{
pid: 4,
name: '商品',
id: 6,
auth: 'product',
},
];
export { authlist };
export default {};
<file_sep>/src/store.js
import Vue from 'vue';
import Vuex from 'vuex';
import { getAuth } from './api';
import { authRouer } from './router';
import user from './user';
import user1 from './user1';
Vue.use(Vuex);
function formatRoute(authlist, routers) {
return routers.filter((router) => {
if (authlist.includes(router.name)) {
// 过滤出有权限的儿子
if (router.children) {
// eslint-disable-next-line no-param-reassign
router.children = formatRoute(authlist, router.children);
}
return true;
}
});
}
export default new Vuex.Store({
strict: process.env.NODE_ENV === 'development',
// 如果不是通过mutations修改state会报警告
state: {
name: '李雷',
loading: false,
hasAuth: false, // 是否请求过路由权限
},
getters: {
xname(state) {
return `小${state.name}`;
},
},
mutations: {
hasAuth(state) {
state.hasAuth = true;
},
hideloading(state) {
state.loading = false;
},
showloading(state) {
state.loading = true;
},
},
actions: {
minus() {
console.log('rootactions');
},
async getRouterList({ commit }) {
// 发送请求 得到权限列表
let authlist = await getAuth();
authlist = authlist.map(item => item.auth);
console.log(authlist);
// 用后台返回的权限对比我们自己的动态路由 确定路由列表
const routerList = formatRoute(authlist, authRouer);
// 修改是否获取权限的状态
commit('hasAuth');
return routerList;
},
hideloading({ commit }) {
commit('hideloading');
},
showloading(store) {
store.commit('showloading');
},
},
modules: {
user,
user1,
},
});
<file_sep>/src/views/vue组件传值.md
# vue 组件传值
1. props 父传子 数字、对象、数组、布尔值、对象的属性 都需要 v-bind: 缩写成 :
2. $emit 和 $on 子传父
```js
//子组件 更新m的值到200 .sync是一种语法糖
this.$emit('update:m', 200);
//父组件 money 和子组件的m值同步
<Son :m.sync="money"></Son>
```
```js
//子组件 v-model 只能针对value
this.$emit('input', 200);
// 父组件通过@value @input的事件进行修改
<Son @value='money'
@input="change"></Son>
// 事件
change(value) {
this.money = value;
}
//可以简写成下面
<Son v-model='money'></Son>
```
3. eventbus 简单项目可以用 eventbus 进行传值
- 把\$bus 变成一个 vue 的实例 同一个 vue 实例
```js
Vue.prototype.$bus = new Vue();
this.$bus.$emit('toS');
this.$bus.$on('toS', () => {
console.log(345);
});
```
<file_sep>/src/user1/acions.js
import * as Types from './mutations-types';
const acions = {
addc({ commit }, payload) {
commit(Types.MADD, payload);
},
};
export default acions;
<file_sep>/src/mock/Icon.md
<Icon type="checkmark"></Icon>
type 图标的名称 String
size 图标的大小,单位是 px Number | String
color 图标的颜色 String<file_sep>/src/lib/axios.js
// lib/axios.js
import axios from 'axios';
import store from '../store';
class AjaxRequest {
// eslint-disable-next-line no-useless-constructor
constructor() {
// 定义一些公共配置
// ajax 请求的地址
this.baseURL = process.env.NODE_ENV === 'development' ? 'http://localhost:3000' : '/';
// ajax 请求超时时间
this.timeout = 2000;
}
merge(options) {
// 合并公共参数和用户传过来的参数
return { baseURL: this.baseURL, timeout: this.timeout, ...options };
}
// eslint-disable-next-line class-methods-use-this
setInterceptors(instance) {
// 设置响应拦截器
instance.interceptors.response.use(
(res) => {
// 让loading 隐藏
console.log('请求完成之后');
store.dispatch('hideloading');
return res.data;
},
err => Promise.reject(err),
);
// 设置请求拦截器
instance.interceptors.request.use(
(config) => {
// 让loading显示
store.dispatch('showloading');
// eslint-disable-next-line no-param-reassign
config.headers.authorization = 'token';
return config;
},
err => Promise.reject(err),
);
}
// eslint-disable-next-line class-methods-use-this
request(options) {
// 实现axios的实例调用
const instance = axios.create();
// setInterceptors 设置拦截器的方法
this.setInterceptors(instance);
// opt 就是合并后的参数
const opt = this.merge(options);
return instance(opt);
}
}
export default new AjaxRequest();
// AjaxRequest.request({
// url:'/list',
// data:{
// a:1
// }
// })
<file_sep>/app.js
// eslint-disable-next-line import/no-extraneous-dependencies
const express = require('express');
const cors = require('cors');
const app = express();
app.use(cors());
app.get('/list', (req, res) => {
res.json('hello world');
});
app.listen(3000);
<file_sep>/src/mock/index.js
// mock/index.js
import Mock from 'mockjs';
import { senList, loginApi } from './home';
import { authlist } from './routerauth';
// 配置ajax请求延时时间
Mock.setup({
timeout: 500,
});
// 正则
Mock.mock(/\/list/, senList);
Mock.mock(/\/login/, loginApi);
Mock.mock(/\/auth/, authlist);
| 8125bc126b3cb9fd4acff20af5510dd2e616fc7f | [
"JavaScript",
"Markdown",
"Shell"
] | 19 | JavaScript | 2422002753/8.16 | ba44fbacc62691c8cc2cb1be3cc359dd40f79a9d | f774a0ea8b07255d497122d461d50980929f23b1 | |
refs/heads/main | <repo_name>jungle8884/community<file_sep>/src/main/java/tech/turl/community/controller/LikeController.java
package tech.turl.community.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import tech.turl.community.entity.Event;
import tech.turl.community.entity.User;
import tech.turl.community.event.EventProducer;
import tech.turl.community.service.LikeService;
import tech.turl.community.util.CommunityConstant;
import tech.turl.community.util.CommunityUtil;
import tech.turl.community.util.HostHolder;
import tech.turl.community.util.RedisKeyUtil;
import java.util.HashMap;
import java.util.Map;
/**
* @author zhengguohuang
* @date 2021/03/21
*/
@Controller
public class LikeController implements CommunityConstant {
@Autowired private HostHolder hostHolder;
@Autowired private LikeService likeService;
@Autowired private EventProducer eventProducer;
@Autowired private RedisTemplate redisTemplate;
/**
* 点赞
*
* @param entityType
* @param entityId
* @return
*/
@PostMapping("/like")
@ResponseBody
public String like(int entityType, int entityId, int entityUserId, int postId) {
User user = hostHolder.getUser();
// 点赞
likeService.like(user.getId(), entityType, entityId, entityUserId);
// 数量
long likeCount = likeService.findEntityLikeCount(entityType, entityId);
// 状态
int likeStatus = likeService.findEntityLikeStatus(user.getId(), entityType, entityId);
// 返回的结果
Map<String, Object> map = new HashMap<>(16);
map.put("likeCount", likeCount);
map.put("likeStatus", likeStatus);
// 触发事件
if (likeStatus == 1) {
Event event =
new Event()
.setTopic(TOPIC_LIKE)
.setUserId(user.getId())
.setEntityType(entityType)
.setEntityId(entityId)
.setEntityUserId(entityUserId)
.setData("postId", postId);
eventProducer.fireEvent(event);
}
if (entityType == ENTITY_TYPE_POST) {
// 计算帖子的分数
String redisKey = RedisKeyUtil.getPostScoreKey();
redisTemplate.opsForSet().add(redisKey, postId);
}
return CommunityUtil.getJSONString(0, null, map);
}
}
<file_sep>/src/main/java/tech/turl/community/service/DataService.java
package tech.turl.community.service;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.connection.RedisStringCommands;
import org.springframework.data.redis.core.RedisCallback;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.stereotype.Service;
import tech.turl.community.util.RedisKeyUtil;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.Date;
import java.util.List;
/**
* @author zhengguohuang
* @date 2021/03/29
*/
@Service
public class DataService {
@Autowired private RedisTemplate redisTemplate;
private SimpleDateFormat df = new SimpleDateFormat("yyyyMMdd");
/**
* 统计UV,将指定ip计入UV
*
* @param ip
*/
public void recordUV(String ip) {
String redisKey = RedisKeyUtil.getUVKey(df.format(new Date()));
redisTemplate.opsForHyperLogLog().add(redisKey, ip);
}
/**
* 统计指定日期范围内的UV
*
* @param startDate
* @param endDate
* @return
*/
public long calculateUV(Date startDate, Date endDate) {
if (startDate == null || endDate == null) {
throw new IllegalArgumentException("参数不能为空");
}
// 整理该日期范围内的Key
List<String> keyList = new ArrayList<>();
Calendar calendar = Calendar.getInstance();
calendar.setTime(startDate);
while (!calendar.getTime().after(endDate)) {
String key = RedisKeyUtil.getUVKey(df.format(calendar.getTime()));
keyList.add(key);
calendar.add(Calendar.DATE, 1);
}
// 合并这些数据
String redisKey = RedisKeyUtil.getUVKey(df.format(startDate), df.format(endDate));
redisTemplate.opsForHyperLogLog().union(redisKey, keyList.toArray());
// 统计数据
return redisTemplate.opsForHyperLogLog().size(redisKey);
}
/**
* 统计DAU,将指定用户计入DAU
*
* @param
*/
public void recordDAU(int userId) {
String redisKey = RedisKeyUtil.getDAUKey(df.format(new Date()));
redisTemplate.opsForValue().setBit(redisKey, userId, true);
}
/**
* 统计指定日期范围内的DAU
*
* @param startDate
* @param endDate
* @return
*/
public long calculateDAU(Date startDate, Date endDate) {
// 整理该日期范围内的Key
List<byte[]> keyList = new ArrayList<>();
Calendar calendar = Calendar.getInstance();
calendar.setTime(startDate);
while (!calendar.getTime().after(endDate)) {
String key = RedisKeyUtil.getDAUKey(df.format(calendar.getTime()));
keyList.add(key.getBytes());
calendar.add(Calendar.DATE, 1);
}
// 合并这些数据
String redisKey = RedisKeyUtil.getDAUKey(df.format(startDate), df.format(endDate));
return (long)
redisTemplate.execute(
(RedisCallback)
redisConnection -> {
redisConnection.bitOp(
RedisStringCommands.BitOperation.OR,
redisKey.getBytes(),
keyList.toArray(new byte[0][0]));
return redisConnection.bitCount(redisKey.getBytes());
});
}
}
<file_sep>/src/main/java/tech/turl/community/controller/SearchController.java
package tech.turl.community.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import tech.turl.community.entity.DiscussPost;
import tech.turl.community.entity.Page;
import tech.turl.community.service.ElasticsearchService;
import tech.turl.community.service.LikeService;
import tech.turl.community.service.UserService;
import tech.turl.community.util.CommunityConstant;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* @author zhengguohuang
* @date 2021/03/26
*/
@Controller
public class SearchController implements CommunityConstant {
@Autowired private ElasticsearchService elasticsearchService;
@Autowired private UserService userService;
@Autowired private LikeService likeService;
/**
* 搜索帖子
*
* @param keyword
* @param page
* @param model
* @return
*/
@GetMapping("/search")
public String search(String keyword, Page page, Model model) {
// 搜索帖子
org.springframework.data.domain.Page<DiscussPost> searchResult =
elasticsearchService.searchDiscussPost(
keyword, page.getCurrent() - 1, page.getLimit());
// 聚合数据
List<Map<String, Object>> discussPosts = new ArrayList<>();
if (searchResult != null) {
for (DiscussPost post : searchResult) {
Map<String, Object> map = new HashMap<>(16);
// 帖子
map.put("post", post);
// 作者
map.put("user", userService.findUserById(post.getUserId()));
// 点赞数量
map.put(
"likeCount",
likeService.findEntityLikeCount(ENTITY_TYPE_POST, post.getId()));
discussPosts.add(map);
}
}
model.addAttribute("discussPosts", discussPosts);
model.addAttribute("keyword", keyword);
page.setPath("/search?keyword=" + keyword);
page.setRows(searchResult == null ? 0 : (int) searchResult.getTotalElements());
return "site/search";
}
}
<file_sep>/src/main/java/tech/turl/community/annotation/LoginRequired.java
package tech.turl.community.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/**
* @author zhengguohuang
* @date 2021/03/16
*/
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface LoginRequired {
}
<file_sep>/README.md
# 仿牛客网社区项目
  
## 项目简介
一个仿照牛客网实现的讨论区,不仅实现了基本的注册、登录、发帖、评论、回复、私信等功能,同时使用前缀树实现敏感词过滤;使用 Redis 实现点赞与关注;使用 Kafka 处理发送评论、点赞和关注等系统通知;使用 Elasticsearch 实现全文搜索,关键词高亮显示;使用 wkhtmltopdf 生成长图和 PDF;实现网站 UV 和 DAU 统计;并将用户头像等信息存于七牛云服务器。
## 在线演示地址
~~http://community.turl.tech~~
## 测试账号
| 用户类型 | 用户名 | 密码 |
| -------- | ------ | ------ |
| 普通用户 | user | 123456 |
| 版主 | banzhu | 123456 |
| 管理员 | admin | 123456 |
## 功能列表
- [x] 邮件发送
- [x] 注册
- [x] 验证码
- [x] 登录
- [ ] 登录出错限制
- [ ] 第三方登录
- [x] 修改头像
- [x] 修改密码
- [x] 敏感词过滤
- [x] 发布帖子
- [x] 我的帖子
- [x] 帖子详情
- [ ] 浏览量
- [x] 评论
- [x] 私信
- [x] 统一异常处理
- [x] 统一日志处理
- [x] 点赞
- [x] 关注
- [ ] 收藏
- [ ] 我的收藏
- [x] 系统通知
- [x] 搜索
- [x] 权限控制
- [x] 置顶、加精、删除
- [x] 网站统计
- [x] 定时执行任务计算热门帖子
- [x] 生成长图
- [x] 文件上传至七牛云
- [x] 监控
## 功能简介
- 使用 Redis 的 set 实现点赞,zset 实现关注,并使用 Redis 存储登录ticket和验证码,解决分布式 Session 问题,使用 Redis 的高级数据类型 HyperLogLog 统计 UV(Unique Visitor),使用 Bitmap 统计 DAU(Daily Active User)。
- 使用 Kafka 处理发送评论、点赞、关注等系统通知、将新发布的帖子异步传输至Elasticsearch服务器,并使用事件进行封装,构建了强大的异步消息系统。
- 使用Elasticsearch做全局搜索,增加关键词高亮显示等功能。
- 热帖排行模块,使用本地缓存 Caffeine作为一级缓存和分布式缓存 Redis作为二级缓存构建多级缓存,避免了缓存雪崩,同时使用使用压测工具测试优化前后性能,将 QPS 提升了4倍(7.6/sec -> 33.5/sec),大大提升了网站访问速度。并使用 Quartz 定时更新热帖排行。
- 使用 Spring Security 做权限控制,替代拦截器的拦截控制,并使用自己的认证方案替代 Security 认证流程,使权限认证和控制更加方便灵活。
## 技术栈
| 技术 | 链接 | 版本 |
| --------------- | ------------------------------------------------------------ | -------------- |
| Spring Boot | https://spring.io/projects/spring-boot | 2.4.3 |
| Spring | https://spring.io/projects/spring-framework | 5.3.4 |
| Spring MVC | https://docs.spring.io/spring-framework/docs/current/reference/html/web.html#spring-web | 5.3.4 |
| MyBatis | http://www.mybatis.org/mybatis-3 | 3.5.1 |
| Redis | https://redis.io/ | 5.0.3 |
| Kafka | http://kafka.apache.org/ | 2.7.0 |
| Elasticsearch | https://www.elastic.co/cn/elasticsearch/ | 7.9.3 |
| Spring Security | https://spring.io/projects/spring-security | 5.4.5 |
| Spring Quartz | https://www.baeldung.com/spring-quartz-schedule | 2.3.2 |
| wkhtmltopdf | https://wkhtmltopdf.org | 0.12.6 |
| kaptcha | https://github.com/penggle/kaptcha | 2.3.2 |
| Thymeleaf | https://www.thymeleaf.org/ | 3.0.12.RELEASE |
| MySQL | https://www.mysql.com/ | 5.7.17 |
| JDK | https://www.oracle.com/java/technologies/javase-downloads.html | 1.8 |
## 系统架构


## 数据库初始化
```sql
create database community;
use community;
source /path/to/sql/init_schema.sql;
source /path/to/sql/init_data.sql;
source /path/to/sql/tables_mysql_innodb.sql;
```
## 运行
1. 安装JDK,Maven
2. 克隆代码到本地
```bash
git clone https://github.com/zhengguohuang/community.git
```
3. 运行打包命令
```bash
mvn package
```
4. 运行项目
```bash
java -jar xxx.jar
```
5. 访问项目
```
http://localhost:8080
```
## 运行效果展示
#### 发帖

#### 帖子详情、评论

#### 私信

#### 系统通知

#### 搜索

#### 网站统计

## 文档
https://easydoc.top/doc/70562937/SJuuLoYS/cI3Atvte
欢迎加群交流: 792364202
## 更新日志
* 2021-3-8 创建项目
<file_sep>/src/test/java/tech/turl/community/TestMessageMapper.java
package tech.turl.community;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.ContextConfiguration;
import tech.turl.community.dao.MessageMapper;
import tech.turl.community.entity.Message;
import java.util.List;
/**
* @author zhengguohuang
* @date 2021/03/19
*/
@SpringBootTest
@ContextConfiguration(classes = CommunityApplication.class)
public class TestMessageMapper {
@Autowired
private MessageMapper messageMapper;
@Test
public void test() {
List<Message> list = messageMapper.selectConversations(111, 0, 20);
for (Message message : list) {
System.out.println(message);
}
int count = messageMapper.selectConversationCount(111);
System.out.println(count);
list = messageMapper.selectLetters("111_112", 0, 10);
for (Message message : list) {
System.out.println(message);
}
count = messageMapper.selectLetterCount("111_112");
System.out.println(count);
count = messageMapper.selectLetterUnreadCount(131, "111_131");
System.out.println(count);
}
}
<file_sep>/src/main/resources/README.md
sensitive-words.txt为本项目收集的敏感词库,仅限用于合法的、积极向上的敏感词过滤使用,严禁用于从事违反法律法规、危害国家、危害人民、不道德的活动!!!<file_sep>/src/main/java/tech/turl/community/util/HostHolder.java
package tech.turl.community.util;
import org.springframework.stereotype.Component;
import tech.turl.community.entity.User;
/**
* 持有用户信息,用于代替session对象
*
* @author zhengguohuang
* @date 2021/3/15
*/
@Component
public class HostHolder {
private ThreadLocal<User> users = new ThreadLocal<>();
public void setUser(User user) {
users.set(user);
}
public User getUser() {
return users.get();
}
public void clear() {
users.remove();
}
}
<file_sep>/src/test/java/tech/turl/community/WkTests.java
package tech.turl.community;
import java.io.IOException;
/**
* @author zhengguohuang
* @date 2021/03/29
*/
public class WkTests {
public static void main(String[] args) throws IOException {
String cmd =
"e:/wkhtmltopdf/bin/wkhtmltoimage --quality 75 https://baidu.com d:/work/data/wk-images/1.png";
Runtime.getRuntime().exec(cmd);
System.out.println("ok");
}
}
<file_sep>/src/main/java/tech/turl/community/config/ThreadPoolConfig.java
package tech.turl.community.config;
import org.springframework.context.annotation.Configuration;
import org.springframework.scheduling.annotation.EnableAsync;
import org.springframework.scheduling.annotation.EnableScheduling;
/**
* @author zhengguohuang
* @date 2021/03/29
*/
@Configuration
@EnableScheduling
@EnableAsync
public class ThreadPoolConfig {}
<file_sep>/src/test/java/tech/turl/community/TestLoginTicketMapper.java
package tech.turl.community;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.ContextConfiguration;
import tech.turl.community.dao.LoginTicketMapper;
import tech.turl.community.entity.LoginTicket;
import java.util.Date;
@SpringBootTest
@ContextConfiguration(classes = CommunityApplication.class)
public class TestLoginTicketMapper {
@Autowired
private LoginTicketMapper loginTicketMapper;
@Test
public void testInsert() {
LoginTicket loginTicket = new LoginTicket();
loginTicket.setUserId(1);
loginTicket.setStatus(0);
loginTicket.setTicket("dddd");
loginTicket.setExpired(new Date());
int ret = loginTicketMapper.insertLoginTicket(loginTicket);
System.out.println(ret);
}
@Test
public void testSelectByTicket() {
LoginTicket loginTicket = loginTicketMapper.selectByTicket("dddd");
System.out.println(loginTicket);
}
@Test
public void testUpdateStatus() {
int ret = loginTicketMapper.updateStatus("dddd", 1);
System.out.println(ret);
}
}
<file_sep>/src/main/java/tech/turl/community/controller/AlphaController.java
package tech.turl.community.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.servlet.ModelAndView;
import tech.turl.community.dao.UserMapper;
import tech.turl.community.entity.Demo;
import tech.turl.community.service.AlphaService;
import tech.turl.community.service.DiscussPostService;
import tech.turl.community.util.CommunityUtil;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/** @author zhengguohuang */
@Controller
@RequestMapping("/alpha")
public class AlphaController {
@Autowired AlphaService alphaService;
@Autowired private UserMapper userMapper;
@Autowired private DiscussPostService discussPostService;
@RequestMapping("/hello")
@ResponseBody
public String sayHello() {
return "Hello Spring Boot.";
}
@RequestMapping("/data")
@ResponseBody
public String getData() {
return alphaService.find();
}
/**
* 带参数GET请求 /students?current=1&limit=20 只能处理GET请求
*
* @param current
* @param limit
* @return
*/
@GetMapping("/students")
@ResponseBody
public String getStudents(
@RequestParam(name = "current", required = false, defaultValue = "1") int current,
@RequestParam(name = "limit", required = false, defaultValue = "20") int limit) {
System.out.println(current);
System.out.println(limit);
return "some students";
}
/**
* 路径中带参数 /student/123
*
* @param id
* @return
*/
@RequestMapping(path = "/student/{id}", method = RequestMethod.GET)
@ResponseBody
public String getStudent(@PathVariable("id") int id) {
System.out.println(id);
return "success";
}
/**
* 响应表单请求
*
* @param name
* @param age
* @return
*/
@RequestMapping(path = "/student", method = RequestMethod.POST)
@ResponseBody
public String saveStudent(String name, int age) {
System.out.println(name);
System.out.println(age);
return "success";
}
/**
* 响应HTML数据
*
* @return
*/
@RequestMapping(path = "/teacher", method = RequestMethod.GET)
public ModelAndView getTeacher() {
ModelAndView mav = new ModelAndView();
mav.addObject("name", "张三");
mav.addObject("age", 30);
mav.setViewName("demo/view");
return mav;
}
@RequestMapping(path = "/school", method = RequestMethod.GET)
public String getSchool(Model model) {
model.addAttribute("name", "Beijing");
model.addAttribute("age", 23);
return "demo/view";
}
/**
* 响应JSON数据(异步请求) Java对象 -> JSON字符串 -> JS对象
*
* @return
*/
@RequestMapping(path = "/emp", method = RequestMethod.GET)
@ResponseBody
public Map<String, Object> getEmp() {
Map<String, Object> emp = new HashMap<>(16);
emp.put("name", "张三");
emp.put("age", 23);
emp.put("salary", 3000);
return emp;
}
@RequestMapping(path = "/emps", method = RequestMethod.GET)
@ResponseBody
public List<Map<String, Object>> getEmps() {
List<Map<String, Object>> list = new ArrayList<>();
Map<String, Object> emp = new HashMap<>(16);
emp.put("name", "张三");
emp.put("age", 23);
emp.put("salary", 3000);
list.add(emp);
emp = new HashMap<>(16);
emp.put("name", "李四");
emp.put("age", 24);
emp.put("salary", 4000);
list.add(emp);
emp = new HashMap<>(16);
emp.put("name", "John");
emp.put("age", 23);
emp.put("salary", 3000);
list.add(emp);
return list;
}
@GetMapping("/cookie/set")
@ResponseBody
public String setCookie(HttpServletResponse response) {
// 创建cookie
Cookie cookie = new Cookie("code", CommunityUtil.generateUUID());
// 设置cookie生效范围
cookie.setPath("/alpha");
// 设置cookie的生存时间
cookie.setMaxAge(60 * 10);
// 发送cookie
response.addCookie(cookie);
return "set cookie";
}
@GetMapping("/cookie/get")
@ResponseBody
public String getCookie(@CookieValue("code") String code) {
System.out.println(code);
return "get cookie";
}
@GetMapping("/session/set")
@ResponseBody
public String setSession(HttpSession session) {
session.setAttribute("id", 1);
session.setAttribute("name", "hzg");
return "set session";
}
@GetMapping("/session/get")
@ResponseBody
public String getSession(HttpSession session) {
System.out.println(session.getAttribute("id"));
System.out.println(session.getAttribute("name"));
return "get cookie";
}
@GetMapping("/demo")
@ResponseBody
public Demo demo() {
Demo demo = new Demo();
demo.setAge(1);
demo.setId(1);
demo.setName("jjj");
return demo;
}
@PostMapping("/ajax")
@ResponseBody
public String testAjax(String name, int age) {
System.out.println(name);
System.out.println(age);
return CommunityUtil.getJSONString(0, "操作成功!");
}
}
<file_sep>/src/test/java/tech/turl/community/CaffeineTests.java
package tech.turl.community;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.ContextConfiguration;
import tech.turl.community.entity.DiscussPost;
import tech.turl.community.service.DiscussPostService;
import java.util.Date;
/**
* @author zhengguohuang
* @date 2021/03/30
*/
@SpringBootTest
@ContextConfiguration(classes = CommunityApplication.class)
public class CaffeineTests {
@Autowired private DiscussPostService postService;
@Test
public void initDataForTest() {
for (int i = 0; i < 100000; i++) {
DiscussPost post = new DiscussPost();
post.setUserId(111);
post.setTitle("互联网秋招暖春计划");
post.setContent("xxxxxxxxxxxxxxxxxxxxx互联网XXX");
post.setCreateTime(new Date());
post.setScore(Math.random() * 2000);
postService.addDiscussPost(post);
}
}
@Test
public void testCache() {
System.out.println(postService.findDiscussPosts(0, 0, 10, 1));
System.out.println(postService.findDiscussPosts(0, 0, 10, 1));
System.out.println(postService.findDiscussPosts(0, 0, 10, 1));
System.out.println(postService.findDiscussPosts(0, 0, 10, 0));
}
}
<file_sep>/src/main/java/tech/turl/community/service/ElasticsearchService.java
package tech.turl.community.service;
import org.elasticsearch.index.query.QueryBuilders;
import org.elasticsearch.search.fetch.subphase.highlight.HighlightBuilder;
import org.elasticsearch.search.sort.SortBuilders;
import org.elasticsearch.search.sort.SortOrder;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.PageImpl;
import org.springframework.data.domain.PageRequest;
import org.springframework.data.domain.Pageable;
import org.springframework.data.elasticsearch.core.ElasticsearchRestTemplate;
import org.springframework.data.elasticsearch.core.SearchHit;
import org.springframework.data.elasticsearch.core.SearchHits;
import org.springframework.data.elasticsearch.core.query.NativeSearchQuery;
import org.springframework.data.elasticsearch.core.query.NativeSearchQueryBuilder;
import org.springframework.stereotype.Service;
import tech.turl.community.dao.elasticsearch.DiscussPostRepository;
import tech.turl.community.entity.DiscussPost;
import java.util.ArrayList;
import java.util.List;
/**
* @author zhengguohuang
* @date 2021/03/26
*/
@Service
public class ElasticsearchService {
@Autowired private DiscussPostRepository discussPostRepository;
@Autowired private ElasticsearchRestTemplate elasticsearchRestTemplate;
/**
* 插入或修改帖子
*
* @param post
*/
public void saveDiscussPost(DiscussPost post) {
discussPostRepository.save(post);
}
/**
* 删除帖子
*
* @param id
*/
public void deleteDiscussPost(int id) {
discussPostRepository.deleteById(id);
}
/**
* 搜索并高亮分页显示
*
* @param keyword
* @param current
* @param limit
* @return
*/
public Page<DiscussPost> searchDiscussPost(String keyword, int current, int limit) {
Pageable pageable = PageRequest.of(current, limit);
NativeSearchQuery searchQuery =
new NativeSearchQueryBuilder()
.withQuery(QueryBuilders.multiMatchQuery(keyword, "title", "content"))
.withSort(SortBuilders.fieldSort("type").order(SortOrder.DESC))
.withSort(SortBuilders.fieldSort("score").order(SortOrder.DESC))
.withSort(SortBuilders.fieldSort("createTime").order(SortOrder.DESC))
.withPageable(pageable)
.withHighlightFields(
new HighlightBuilder.Field("title")
.preTags("<em>")
.postTags("</em>"),
new HighlightBuilder.Field("content")
.preTags("<em>")
.postTags("</em>"))
.build();
SearchHits<DiscussPost> searchHits =
elasticsearchRestTemplate.search(searchQuery, DiscussPost.class);
if (searchHits.getTotalHits() <= 0) {
return null;
}
List<DiscussPost> list = new ArrayList<>();
for (SearchHit<DiscussPost> hit : searchHits) {
DiscussPost content = hit.getContent();
DiscussPost post = new DiscussPost();
BeanUtils.copyProperties(content, post);
List<String> list1 = hit.getHighlightFields().get("title");
if (list1 != null) {
post.setTitle(list1.get(0));
}
List<String> list2 = hit.getHighlightFields().get("content");
if (list2 != null) {
post.setContent(list2.get(0));
}
list.add(post);
}
return new PageImpl<>(list, pageable, searchHits.getTotalHits());
}
}
<file_sep>/src/main/java/tech/turl/community/dao/LoginTicketMapper.java
package tech.turl.community.dao;
import org.apache.ibatis.annotations.*;
import tech.turl.community.entity.LoginTicket;
/**
* @author zhengguohuang
* @date 2021/3/15
*/
@Mapper
@Deprecated
public interface LoginTicketMapper {
/**
* 插入login_ticket表
*
* @param loginTicket
* @return
*/
@Insert({
"insert into login_ticket(user_id, ticket, status, expired) ",
"values(#{userId}, #{ticket}, #{status}, #{expired})"
})
@Options(useGeneratedKeys = true, keyProperty = "id")
int insertLoginTicket(LoginTicket loginTicket);
/**
* 通过Ticket查找记录
*
* @param ticket
* @return
*/
@Select({
"select id, user_id, ticket, status, expired ",
"from login_ticket where ticket=#{ticket}"
})
LoginTicket selectByTicket(String ticket);
/**
* 更新login_ticket的status字段
*
* @param ticket
* @param status
* @return
*/
@Update({
"update login_ticket set status = #{status} where ticket=#{ticket} "
})
int updateStatus(@Param("ticket") String ticket, @Param("status") int status);
}
| 2d1b0441a9fafb534c6dc8b573e50894bf57edee | [
"Markdown",
"Java"
] | 15 | Java | jungle8884/community | b697457b81c1cf3bde15e82e98fea997444c5ccb | 10b75e208e63b9c9ae1691bb18f1e3d03784a5ce | |
refs/heads/master | <file_sep>## Kedge Plugin for Helm
#### Using the plugin -
1. Install the plugin
`helm plugin install https://github.com/kedgeproject/kedge-helm-plugin.git`
2. Package the chart using
`helm kedge package <path to chart>`
An example Kedge application is located at examples/wordpress/kedge/
Run,
`helm kedge package examples/wordpress/kedge/`
The plugin generates Kubernetes artifacts and places in examples/wordpress/templates/kedge-generated.yaml
3. Deploy the generated chart
`helm install wordpress-0.1.0.tgz`
#### Short PoC:
[](https://asciinema.org/a/dmGQDj0X89S3YCrYZ2oPEHF7G)
##### What's next?
- Add support for parameterization
<file_sep># Wordpress
Deploy a Wordpress container with a database (MariaDB) as well as secrets using Kedge!
In all, we will define a:
- service
- secret
- configmap
- deployment
# How to deploy
1. Download the files
```sh
wget https://raw.githubusercontent.com/kedgeproject/kedge/master/examples/wordpress/wordpress.yaml
wget https://raw.githubusercontent.com/kedgeproject/kedge/master/examples/wordpress/mariadb.yaml
```
2. Deploy using `kedge`
```sh
$ kedge apply -f wordpress.yaml -f mariadb.yaml
persistentvolumeclaim "database" created
service "database" created
secret "database-root-password" created
secret "database-user-password" created
configmap "database" created
deployment "database" created
service "wordpress" created
deployment "wordpress" created
```
3. Access your Wordpress instance
If you are using `minikube` for local Kubernetes deployment, you can access your Wordpress instance using `minikube service`
```sh
$ minikube service wordpress
Opening kubernetes service default/wordpress in default browser...
```
<file_sep>Helm chart defined using the Kedge spec
<file_sep>#!/bin/bash
set -eu
usage() {
cat << EOF
This plugin provides integration with the kedge project.
Available Commands:
build Build the Kedge files into Kubernetes artifacts, but don't package.
package Generated a packaged Helm chart.
Typical usage:
$ helm create mychart
$ mkdir -p mychart/kedge
$ place kedge files in mychart/kedge/
$ helm kedge package mychart
$ helm install ./mychart-0.1.0.tgz
EOF
}
build() {
kedge generate -f $1/kedge/ > $1/templates/kedge-generated.yaml
}
package() {
build $1
helm package $1
}
if [[ $# < 1 ]]; then
echo "===> ERROR: Subcommand required. Try 'helm kedge help'"
exit 1
elif [[ $# < 2 && $1 != "help" ]]; then
echo "===> ERROR: Missing chart path. Use '.' for the present directory."
exit 1
fi
case "${1:-"help"}" in
"package")
package $2
;;
"show")
show $2
;;
"build")
build $2
;;
"help")
usage
;;
*)
echo $1
usage
exit 1
;;
esac
| f29a6b22607ebc43242e965e03b75938134d818d | [
"Markdown",
"Text",
"Shell"
] | 4 | Markdown | kedgeproject/kedge-helm-plugin | 766fdb20f0e5a05b3d01f0427f3e8b99ccc88cf5 | c8bae2f4472d6c3664233534e71c47ca7647f271 | |
refs/heads/master | <file_sep>package ir.saafta.repo;
import ir.saafta.model.Feedback;
import ir.saafta.model.User;
import org.springframework.data.repository.PagingAndSortingRepository;
/**
* Created by reza on 11/14/16.
*/
public interface FeedbackRepository extends PagingAndSortingRepository<Feedback, Long> {
void deleteAllByUser(User user);
}
<file_sep>(function () {
'use strict';
angular
.module('app')
.config(config);
/** @ngInject */
function config($qProvider, $logProvider, $httpProvider, $provide,
ADMdtpProvider, moment) {
// Enable log
$logProvider.debugEnabled(true);
console.log('$qProvider.errorOnUnhandledRejections', $qProvider.errorOnUnhandledRejections);
$qProvider.errorOnUnhandledRejections(false);
// @desc add $http Interceptor
// $httpProvider.interceptors.push('authInterceptor');
$httpProvider.defaults.withCredentials = true;
// disable # in url
// $locationProvider.html5Mode(true);
/////////////////////////////////
// Set options third-party lib //
/////////////////////////////////
ADMdtpProvider.setOptions({
autoClose: true,
calType: 'jalali',
dtpType: 'date',
format: 'YYYY-MM-DD'
});
// Set toastr options
/*angular.extend(toastrConfig, {
allowHtml: true,
closeButton: true,
closeHtml: '<button class="pull-left">×</button>',
extendedTimeOut: 500,
positionClass: 'toast-top-right',
preventDuplicates: true,
progressBar: true,
tapToDismiss: true,
target: 'body',
timeOut: 3000
});
*/
//moment
moment.loadPersian();
$provide.decorator('uibTabsetDirective', function ($delegate) {
$delegate[0].templateUrl = "assets/tpl/uib-tabset-app-kit.html";
$delegate[0].scope['tabsetClass'] = '@';
return $delegate;
});
$provide.decorator('uibTabDirective', function ($delegate) {
$delegate[0].templateUrl = "assets/tpl/uib-tab-app-kit.html";
$delegate[0].scope['tabIconClass'] = '@';
return $delegate;
});
$provide.decorator('uibAlertDirective', function ($delegate) {
$delegate[0].templateUrl = "assets/tpl/uib-alert-app-kit.html";
return $delegate;
});
}
})();
<file_sep>(function () {
'use strict';
/**
* @ngdoc directive
* @name app.directive:sidebar
* @description
* # sidebar
*/
angular
.module('app')
.directive('sidebar', sidebar);
/** @ngInject */
function sidebar() {
return {
templateUrl: 'app/main/sidebar/sidebar.html',
restrict: 'E',
replace: true,
controller: SidebarCtrl,
controllerAs: 'vm',
bindToController: true // because the scope is isolated
};
/** @ngInject */
function SidebarCtrl(sidebarService, $log, principal) {
/* jshint validthis: true */
var vm = this;
vm.selectedMenu = 'dashboard';
vm.collapseVar = 0;
vm.multiCollapseVar = 0;
var user = {};
principal.identity().then(function (data) {
user = data;
vm.user = data;
});
vm.check = check;
vm.multiCheck = multiCheck;
vm.hasAccess = hasAccess;
activate();
function activate() {
$log.info('Sidebar module activated');
sidebarService.getSidebar().then(
function (data) {
vm.data = data;
}
);
}
function check(x) {
if (x === vm.collapseVar) {
vm.collapseVar = 0;
}
else {
vm.collapseVar = x;
}
}
function multiCheck(y) {
if (y === vm.multiCollapseVar) {
vm.multiCollapseVar = 0;
}
else {
vm.multiCollapseVar = y;
}
}
function hasAccess(authorities) {
if (!authorities || !authorities.length) {
return true;
}
else if (!user.authorities || !user.authorities.length) {
return false;
}
for (var i = 0; i < authorities.length; i++) {
for (var j = 0; j < user.authorities.length; j++) {
if (authorities[i] === user.authorities[j].authority) {
return true;
}
}
}
return false;
}
}
}
})();
<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('AccessController', AccessController);
/** @ngInject */
function AccessController($log, NgTableParams, accessService, toastr, $ngConfirm, $scope) {
var vm = this;
vm.title = 'AccessController';
vm.accessTable = new NgTableParams({
page: 1,
count: 20
}, {
getData: getAccesses
});
vm.delete = Delete;
activate();
////////////////
function activate() {
$log.debug('Access module activated');
}
function getAccesses(tabelparams) {
var params = {
page: tabelparams.page() - 1,
size: tabelparams.count()
};
return accessService.getAccesses(params).then(function (data) {
tabelparams.total(data.totalElements);
return data.content;
});
}
function Delete(id) {
$ngConfirm({
title: 'حذف دسترسی',
content: '<span class="text-danger">{{"Are you sure about delete this item?"|translate}}</span>',
scope: $scope,
buttons: {
sayBoo: {
text: 'بله',
btnClass: 'btn-blue',
action: function (scope, button) {
accessService.deleteAccess(id)
.then(function () {
toastr.success('دسترسی با موفقیت حذف شد.');
vm.accessTable.reload();
}, function () {
toastr.error('خطا در حذف دسترسی!');
});
return true;
}
},
somethingElse: {
text: 'خیر',
btnClass: 'btn-orange',
action: function (scope, button) {
return true;
}
}
}
});
}
}
})();<file_sep>package ir.saafta.repo;
import ir.saafta.model.Content;
import org.springframework.data.repository.PagingAndSortingRepository;
/**
* Created by r.kashmir on 7/16/2017.
*/
public interface ContentRepository extends PagingAndSortingRepository<Content, Long> {
}
<file_sep>package ir.saafta.web;
import ir.saafta.model.Event;
import ir.saafta.repo.EventRepository;
import ir.saafta.repo.EventSpec;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.security.access.prepost.PreAuthorize;
import org.springframework.web.bind.annotation.*;
/**
* Created by Reza on 11/18/2016.
*/
@RestController
@RequestMapping("/event")
public class EventController {
private final EventRepository eventRepository;
@Autowired
public EventController(EventRepository eventRepository) {
this.eventRepository = eventRepository;
}
@PreAuthorize("hasAuthority('admin')")
@RequestMapping(value = "/{id}", method = RequestMethod.GET)
public Event getById(@PathVariable Long id) {
return eventRepository.findOne(id);
}
@PreAuthorize("hasAuthority('admin')")
@RequestMapping(method = RequestMethod.PUT)
public Page<Event> getEvents(Pageable pageable, @RequestBody EventSpec eventSpec) {
return eventRepository.findAll(eventSpec, pageable);
}
}
<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('CategoryController', CategoryController);
/** @ngInject */
function CategoryController($log, homeService, host, $timeout, $uibModal) {
var vm = this;
vm.title = 'CategoryController';
vm.dashboard = {};
activate();
vm.slides = [
{id: 0},
{id: 1},
{id: 2},
{id: 3},
{id: 4},
{id: 5},
{id: 6}
];
vm.host = host;
vm.getStarClass = getStarClass;
vm.myInterval = 3000;
vm.noWrapSlides = false;
vm.responsiveSetting = [
{
breakpoint: 964,
settings: {
slidesToShow: 2,
slidesToScroll: 2,
dots: true
}
},
{
breakpoint: 768,
settings: {
slidesToShow: 1,
slidesToScroll: 1,
dots: true
}
}
];
////////////////
function activate() {
$log.debug('Category module activated');
getSpecifics();
}
function getSpecifics() {
homeService.getSpecificApps().then(function (data) {
vm.specificApps = data;
//TODO: remove it before commit
if (vm.specificApps.length < 7)
vm.specificApps = vm.specificApps.concat(data);
if (vm.specificApps.length < 7)
vm.specificApps = vm.specificApps.concat(data);
$timeout(function () {
var width = $('.slick-slide').css("width");
/*if(width) {
width = parseInt(width.substring(0, width.length - 2));
console.log(width);
if (width < 80) {
$('.slick-slide').css("width", "80px")
}
}*/
}, 5000)
})
}
function getStarClass(app, index) {
if (app.rating - index > 1) {
return "star";
}
else if (app.rating - index > 0) {
return "star-half-o";
} else {
return "star-o";
}
}
}
})();<file_sep>/**
* Created by reza on 11/14/16.
*/
(function () {
'use strict';
angular
.module('app')
.config(routeConfig);
/** @ngInject */
function routeConfig($stateProvider) {
$stateProvider
.state('root.comment', {
controller: 'CommentController',
controllerAs: 'vm',
data: {
roles: [
"admin"
]
},
resolve: {},
templateUrl: 'app/components/comment/list-comment.html',
url: '/comment'
})
.state('root.createUpdateComment', {
controller: 'CreateUpdateCommentController',
controllerAs: 'vm',
data: {
roles: [
"admin"
]
},
resolve: {},
templateUrl: 'app/components/comment/create-update-comment.html',
url: '/comment/:method?id'
})
;
}
})();
<file_sep>(function () {
'use strict';
angular
.module('app')
.run(runBlock);
/** @ngInject */
function runBlock($log, $confirmModalDefaults, $window, $rootScope, ngTableDefaults, principal, $transitions) {
//set confirm default
$confirmModalDefaults.templateUrl = 'app/directives/confirm/confirm-tpl.html';
$confirmModalDefaults.defaultLabels.title = '';
$confirmModalDefaults.defaultLabels.ok = 'بله';
$confirmModalDefaults.defaultLabels.cancel = 'خیر';
$confirmModalDefaults.size = 'sm';
//ng-table default
ngTableDefaults.params.count = 20;
ngTableDefaults.settings.counts = [10, 20, 40, 60, 80, 100];
ngTableDefaults.settings.paginationMaxBlocks = 5;
ngTableDefaults.settings.paginationMinBlocks = 2;
$transitions.onStart({to: '**'}, function (trans) {
console.log('stateChangeStart:', trans.$to());
if (trans.$to().name !== 'login') {
// principal.authorize();
}
});
$transitions.onSuccess({to: '**'}, function (trans) {
console.log('sstateChangeSuccess;');
$window.scrollTo(0, 0);
});
$log.debug('runBlock end');
}
})();
<file_sep>(function () {
'use strict';
angular
.module('app')
.directive('fileUpload', fileUpload);
/** @ngInject */
function fileUpload() {
var directive = {
restrict: 'E',
scope: {
accept: '@',
file: '=',
label: '@',
uploading: '='
},
templateUrl: 'app/directives/file-upload/file-upload.html'
};
return directive;
}
})();
<file_sep>package ir.saafta.web;
import com.netflix.hystrix.exception.HystrixBadRequestException;
import ir.saafta.model.Category;
import ir.saafta.model.Picture;
import ir.saafta.repo.CategoryRepository;
import ir.saafta.repo.PictureRepository;
import org.omg.SendingContext.RunTimeOperations;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.security.access.prepost.PreAuthorize;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
import java.text.ParseException;
/**
* Created by reza on 11/12/16.
*/
@RestController
@RequestMapping("/category")
public class CategoryController {
private final Logger logger = LoggerFactory.getLogger(this.getClass());
private final CategoryRepository categoryRepository;
private final PictureRepository pictureRepository;
@Autowired
public CategoryController(CategoryRepository categoryRepository, PictureRepository pictureRepository) {
this.categoryRepository = categoryRepository;
this.pictureRepository = pictureRepository;
}
@PreAuthorize("hasAuthority('admin')")
@RequestMapping(value = "/", method = RequestMethod.POST, consumes = "multipart/*")
public Category create(MultipartFile pic, String name) throws IOException, ParseException {
if (pic == null) {
logger.error("no picture data");
throw new HystrixBadRequestException("Picture can not be empty");
}
Picture picture = new Picture(pic.getBytes(), name, "category", pic.getOriginalFilename());
picture = pictureRepository.save(picture);
Category category = new Category();
category.setName(name);
category.setPicture(picture);
return categoryRepository.save(category);
}
@RequestMapping(value = "/{id}", method = RequestMethod.GET)
public Category getOne(@PathVariable("id") Long id) {
return categoryRepository.findOne(id);
}
@RequestMapping(method = RequestMethod.GET)
public Page<Category> getCategoriesList(Pageable pageable) {
return categoryRepository.findAll(pageable);
}
@RequestMapping(value = "/all",method = RequestMethod.GET)
public Iterable<Category> getAllCategories(){
return categoryRepository.findAll();
}
@PreAuthorize("hasAuthority('admin')")
@RequestMapping(value = "/{id}", method = RequestMethod.PUT, consumes = "multipart/*")
public Category update(@PathVariable Long id, MultipartFile pic, String name) throws IOException, ParseException {
Category category = categoryRepository.findOne(id);
Picture picture;
if (pic != null) {
if (category.getPicture() != null) {
picture = pictureRepository.findOne(category.getPicture().getId());
picture.setData(pic.getBytes());
picture.setLabel(name);
picture.setFormat(pic.getOriginalFilename());
} else {
picture = new Picture(pic.getBytes(), name, "category", pic.getOriginalFilename());
}
picture = pictureRepository.save(picture);
if (category.getPicture() == null) {
category.setPicture(picture);
}
}
category.setName(name);
return categoryRepository.save(category);
}
@PreAuthorize("hasAuthority('admin')")
@RequestMapping(value = "/{id}", method = RequestMethod.DELETE)
public void delete(@PathVariable Long id) {
try {
Picture picture = categoryRepository.findOne(id).getPicture();
categoryRepository.delete(id);
pictureRepository.delete(picture);
} catch (org.springframework.dao.DataIntegrityViolationException exception) {
throw new RuntimeException("Category can not be deleted due to depended information");
}
}
}
<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('UserGroupController', UserGroupController);
/** @ngInject */
function UserGroupController($log, NgTableParams, userGroupService, util, $ngConfirm, $scope) {
var vm = this;
vm.title = 'UserGroupController';
vm.userGroupTable = new NgTableParams({
page: 1,
count: 20
}, {
getData: getUserGroups
});
vm.delete = Delete;
vm.update = update;
vm.create = create;
activate();
////////////////
function activate() {
$log.debug('UserGroup module activated');
}
function getUserGroups(tabelparams) {
var params = {
page: tabelparams.page() - 1,
size: tabelparams.count()
};
angular.forEach(tabelparams.sorting(), function (value, key) {
params.sort = key + ',' + value;
});
return userGroupService.getUserGroups(params).then(function (data) {
tabelparams.total(data.totalElements);
return data.content;
}, function (error) {
});
}
function Delete(id) {
$ngConfirm({
title: 'حذف گروه کاربری',
content: '<span class="text-danger">{{"Are you sure about delete this item?"|translate}}</span>',
scope: $scope,
buttons: {
sayBoo: {
text: 'بله',
btnClass: 'btn-blue',
action: function (scope, button) {
vm.loading = true;
userGroupService.deleteUserGroup(id)
.then(function () {
util.toast('success', 'APP.MODULES.USER.MESSAGE.SUCCESS.DELETE_GROUP');
vm.userGroupTable.reload();
vm.loading = false;
}, function () {
util.toast('error', 'APP.MODULES.USER.MESSAGE.FAIL.DELETE_GROUP');
vm.loading = false;
});
return true;
}
},
somethingElse: {
text: 'خیر',
btnClass: 'btn-orange',
action: function (scope, button) {
return true;
}
}
}
});
}
function update(userGroup) {
vm.loading = true;
userGroupService.updateUserGroup(userGroup).then(function () {
util.toast('success', 'APP.MODULES.USER.MESSAGE.SUCCESS.UPDATE_GROUP');
vm.toggleEdit[userGroup.id] = false;
vm.loading = false;
}, function () {
util.toast('error', 'APP.MODULES.USER.MESSAGE.FAIL.UPDATE_GROUP');
vm.loading = false;
})
}
function create() {
vm.loading = true;
userGroupService.createUserGroup(vm.userGroup).then(function () {
util.toast('success', 'APP.MODULES.USER.MESSAGE.SUCCESS.CREATE_GROUP');
vm.toggleCreate = false;
vm.loading = false;
vm.userGroupTable.reload();
}, function () {
util.toast('error', 'APP.MODULES.USER.MESSAGE.FAIL.CREATE_GROUP');
vm.loading = false;
})
}
}
})();<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('UserController', UserController);
/** @ngInject */
function UserController($log, $stateParams, NgTableParams, userService, userGroupService, toastr, $uibModal,
util, principal, $ngConfirm, $scope) {
var vm = this;
vm.title = 'UserController';
vm.groups = [];
vm.delete = Delete;
vm.getCaption = getCaption;
vm.getAuthorityClass = getAuthorityClass;
vm.showSessions = showSessions;
vm.addUserToGroup = addUserToGroup;
vm.remove = remove;
activate();
////////////////
function activate() {
$log.debug('User module activated', $stateParams);
getAuthorities();
getAllUserGroups().then(function (data) {
data.forEach(function (group) {
if ($stateParams.groupId && parseInt(group.id) === parseInt($stateParams.groupId)) {
vm.group = group;
getAllUsers();
}
vm.groups.push({
id: group.id,
title: group.title
})
});
console.log(vm.groups);
vm.userTable = new NgTableParams({
page: 1,
count: 20
}, {
getData: getUsers
});
});
principal.identity().then(function (data) {
vm.me = data;
});
}
function getAuthorities() {
return userService.getAuthorities().then(function (data) {
vm.authorities = data;
});
}
function getAllUserGroups() {
return userGroupService.getAllUserGroups();
}
function getAuthorityClass(authority) {
if (authority === 'admin') {
return "btn-success";
}
return "btn-default";
}
function getCaption(authority) {
if (vm.authorities) {
for (var i = 0; i < vm.authorities.length; i++) {
if (vm.authorities[i].authority === authority) {
return vm.authorities[i].caption;
}
}
}
}
function getUsers(tabelparams) {
var params = {
page: tabelparams.page() - 1,
size: tabelparams.count()
};
if (tabelparams.filter().username) {
params.username = tabelparams.filter().username;
}
if (tabelparams.filter().name) {
params.name = tabelparams.filter().name;
}
if (tabelparams.filter().family) {
params.family = tabelparams.filter().family;
}
if (tabelparams.filter().enabled !== undefined) {
params.enabled = tabelparams.filter().enabled;
}
// console.log('tabelparams.sorting()', tabelparams.sorting());
if (tabelparams.filter().group !== undefined) {
params.groupId = tabelparams.filter().group;
} else if ($stateParams.groupId) {
params.groupId = $stateParams.groupId;
}
angular.forEach(tabelparams.sorting(), function (value, key) {
params.sort = key + ',' + value;
});
return userService.getUsers(params).then(function (data) {
tabelparams.total(data.totalElements);
vm.users = data.content;
return data.content;
}, function () {
vm.users = [];
});
}
function Delete(username) {
$ngConfirm({
title: 'حذف کاربر',
content: '<span class="text-danger">{{"Are you sure about delete this item?"|translate}}</span>',
scope: $scope,
buttons: {
sayBoo: {
text: 'بله',
btnClass: 'btn-blue',
action: function(scope, button){
vm.loading = true;
userService.deleteUser(username).then(function () {
util.toast('success','APP.MODULES.USER.MESSAGE.SUCCESS.DELETE');
vm.userTable.reload();
vm.loading = false;
}, function (error) {
if(error.data.exception === 'java.lang.ArrayStoreException')
util.toast('error', error.data.message);
else
util.toast('error', 'APP.MODULES.USER.MESSAGE.FAIL.DELETE');
vm.loading = false;
});
return true;
}
},
somethingElse: {
text: 'خیر',
btnClass: 'btn-orange',
action: function(scope, button){
return true;
}
}
}
});
/*userService.deleteUser(username).then(function () {
toastr.success('APP.MODULES.USER.MESSAGE.SUCCESS.DELETE');
vm.userTable.reload();
}, function () {
toastr.error('APP.MODULES.USER.MESSAGE.FAIL.DELETE');
});*/
}
function showSessions(user) {
var modalInstance = $uibModal.open({
animation: true,
templateUrl: 'app/components/user/session/sessions.html',
controller: 'SessionsController',
controllerAs: 'sm',
backdrop: 'static',
size: 'lg',
resolve: {
user: function () {
return user;
}
}
});
modalInstance.result.then(function () {
});
}
function getAllUsers() {
userGroupService.getNonGroupUsers($stateParams.groupId).then(function (data) {
vm.allUsers = data;
})
}
function addUserToGroup() {
userGroupService.addUserToGroup(vm.group.id, vm.user.username).then(function (data) {
vm.user = undefined;
vm.allUsers = data;
vm.userTable.reload();
})
}
function remove(username) {
if (vm.group) {
$ngConfirm({
title: 'حذف کاربر از گروه',
content: '<span class="text-danger">{{"Are you sure about delete this item?"|translate}}</span>',
scope: $scope,
buttons: {
sayBoo: {
text: 'بله',
btnClass: 'btn-blue',
action: function (scope, button) {
vm.loading = true;
userGroupService.removeUserFromGroup(vm.group.id, username).then(function (data) {
util.toast('success', 'APP.MODULES.USER.MESSAGE.SUCCESS.REMOVE_USER_FROM_GROUP');
vm.allUsers = data;
vm.userTable.reload();
vm.loading = false;
}, function () {
util.toast('error', 'APP.MODULES.USER.MESSAGE.FAIL.REMOVE_USER_FROM_GROUP');
vm.loading = false;
});
return true;
}
},
somethingElse: {
text: 'خیر',
btnClass: 'btn-orange',
action: function (scope, button) {
return true;
}
}
}
});
}
}
}
})();<file_sep>/**
* Created by reza on 11/14/16.
*/
(function () {
'use strict';
angular
.module('app')
.config(routeConfig);
/** @ngInject */
function routeConfig($stateProvider) {
$stateProvider
.state('root.access', {
controller: 'AccessController',
controllerAs: 'vm',
data: {
roles: [
"admin"
]
},
resolve: {},
templateUrl: 'app/components/access/list-access.html',
url: '/access'
})
.state('root.createUpdateAccess', {
controller: 'CreateUpdateAccessController',
controllerAs: 'vm',
data: {
roles: [
"admin"
]
},
resolve: {},
templateUrl: 'app/components/access/create-update-access.html',
url: '/access/:method?id'
})
;
}
})();
<file_sep>package ir.saafta.repo;
import ir.saafta.model.Attempt;
import org.springframework.data.repository.PagingAndSortingRepository;
public interface AttemptRepository extends PagingAndSortingRepository<Attempt, String> {
}
<file_sep>/**
* Created by reza on 11/14/16.
*/
(function () {
'use strict';
angular
.module('app')
.config(routeConfig);
/** @ngInject */
function routeConfig($stateProvider) {
$stateProvider
.state('root.user', {
controller: 'UserController',
controllerAs: 'vm',
data: {
roles: [
"admin"
]
},
resolve: {},
templateUrl: 'app/components/user/list-user.html',
url: '/user'
})
.state('root.createUpdateUser', {
controller: 'CreateUpdateUserController',
controllerAs: 'vm',
data: {
roles: [
"admin"
]
},
resolve: {},
templateUrl: 'app/components/user/create-update-user.html',
url: '/user/:method/:username'
})
;
}
})();
<file_sep>package ir.saafta.model;
import java.io.Serializable;
/**
* User: <NAME>
*/
@SuppressWarnings("unused")
public class AuthorityPK implements Serializable {
private String username;
private String authority;
public AuthorityPK(String username, String authority) {
this.username = username;
this.authority = authority;
}
public AuthorityPK() {
}
}
<file_sep>apply plugin: 'java'
apply plugin: 'idea'
apply plugin: 'application'
mainClassName = 'ir.saafta.SbazaarApplication'
jar {
baseName = 'sbazaar'
version = '0.0.1-SNAPSHOT'
}
sourceCompatibility = 1.8
targetCompatibility = 1.8
repositories {
mavenCentral()
// mavenLocal()
}
dependencies {
compile('org.springframework.boot:spring-boot-starter-data-jpa:1.4.2.RELEASE')
compile('org.springframework.boot:spring-boot-starter-web:1.4.2.RELEASE')
compile('org.springframework.boot:spring-boot-starter-security:1.4.2.RELEASE')
compile('com.github.mkopylec:recaptcha-spring-boot-starter:1.3.8')
compile('io.jsonwebtoken:jjwt:0.7.0')
compile group: 'mysql', name: 'mysql-connector-java', version: '5.1.40'
compile 'io.springfox:springfox-swagger2:2.6.1'
compile 'io.springfox:springfox-swagger-ui:2.6.1'
compile 'org.springframework.cloud:spring-cloud-starter-zuul:1.2.2.RELEASE'
compile 'org.apache.tika:tika:0.3'
compile group: 'org.apache.tika', name: 'tika-core', version: '1.15'
compile group: 'com.github.cage', name: 'cage', version: '1.0'
compile group: 'org.springframework.session', name: 'spring-session', version: '1.3.3.RELEASE'
compile group: 'com.hazelcast', name: 'hazelcast', version: '3.9.4'
compile group: 'com.github.ulisesbocchio', name: 'jasypt-spring-boot-starter', version: '1.16'
testCompile('org.springframework.boot:spring-boot-starter-test:1.4.2.RELEASE')
}
<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('CreateUpdateCategoryController', CreateUpdateCategoryController);
/** @ngInject */
function CreateUpdateCategoryController($log, $timeout, categoryService, $stateParams, toastr, $state, host) {
var vm = this;
vm.title = 'CreateUpdateUserController';
vm.host = host;
vm.method = $stateParams.method;
vm.category = {
id: $stateParams.id
};
vm.save = save;
vm.reset = reset;
vm.date = new Date();
activate();
////////////////
function activate() {
$log.debug('Category module activated');
getCategory();
}
function save() {
switch (vm.method) {
case 'create':
categoryService.createCategory(vm.category.name, vm.cropper.croppedImage)
.then(function (data) {
$timeout(function () {
$state.go('root.category');
}, 3000);
toastr.success('دستهبندی با موفقیت افزوده شد.');
}, function () {
toastr.error('خطا در ایجاد دستهبندی!');
});
break;
case 'update':
console.log('save ', vm.category);
var croppedImage = vm.cropper ? vm.cropper.croppedImage : null;
categoryService.updateCategory(vm.category.id, vm.category.name, croppedImage)
.then(function (data) {
vm.category = data;
toastr.success('دستهبندی با موفقیت ویرایش شد.');
vm.date = new Date();
vm.cropper = null;
}, function () {
toastr.error('خطا در ویرایش دستهبندی!');
});
break;
}
}
function reset() {
switch (vm.method) {
case 'create':
vm.category = {};
break;
case 'update':
getCategory();
}
}
function getCategory() {
if (vm.category.id) {
categoryService.getCategory(vm.category.id).then(function (data) {
vm.category = data;
});
}
}
}
})();<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('ApplicationController', ApplicationController);
/** @ngInject */
function ApplicationController($log, $q, $stateParams, NgTableParams, applicationService, util, categoryService,
ownerService, userService, userGroupService, host, $ngConfirm, $scope) {
var vm = this;
vm.title = 'ApplicationController';
vm.host = host;
vm.delete = Delete;
vm.statuses = [
{'id': 'DEACTIVATED', 'title': 'غیرفعال'},
{'id': 'PUBLIC', 'title': 'عمومی'},
{'id': 'LAUNCHER', 'title': 'پوسته امن'},
{'id': 'NON_LAUNCHER', 'title': 'پوسته عادی'}
];
activate();
////////////////
function activate() {
$log.debug('Application module activated');
$q.all([getCategories(), getOwners(), getUsers()]).then(function (data) {
vm.categories = data[0];
vm.owners = data[1];
vm.users = data[2];
vm.categories.forEach(function (category) {
category.title = category.name;
});
// vm.categories.push({title: 'انتخاب و جستجو بر اساس دستهبندی...'});
vm.owners.forEach(function (owner) {
owner.title = owner.name;
});
// vm.owners.push({title: 'انتخاب و جستجو بر اساس شرکت...'});
vm.users.forEach(function (user) {
user.title = user.completeName + ' (' + user.username + ')';
user.id = user.username;
});
// vm.users.push({title: 'انتخاب و جستجو بر اساس کاربر...'});
console.log('vm.categories', vm.categories);
vm.applicationTable = new NgTableParams({
page: 1,
count: 20
}, {
getData: getApplications
});
});
getGroup();
}
function getApplications(tabelparams) {
// console.log(tabelparams.filter());
var params = {
page: tabelparams.page() - 1,
size: tabelparams.count()
};
var data = {};
if(tabelparams.filter().name)
data.name = tabelparams.filter().name;
if (tabelparams.filter().status)
data.statuses = [tabelparams.filter().status];
if(tabelparams.filter().appPackage)
data.appPackage = tabelparams.filter().appPackage;
if(tabelparams.filter().fee)
data.fee = tabelparams.filter().fee;
if(tabelparams.filter().category)
data.category = {id: tabelparams.filter().category};
if (tabelparams.filter().owner)
data.ownerId = tabelparams.filter().owner;
if(tabelparams.filter().user)
data.username = tabelparams.filter().user;
if (tabelparams.filter().disabled !== undefined)
data.disabled = tabelparams.filter().disabled;
if ($stateParams.groupId)
data.group = {id: $stateParams.groupId}
return applicationService.getApplications(data, params).then(function (data) {
data.content.forEach(function (app) {
app.user = vm.users.filter(function (user) {
return user.username === app.userId;
})[0];
app.owner = vm.owners.filter(function (owner) {
return owner.id === app.ownerId;
})[0];
app.installationCount = app.installationCount || 0;
});
tabelparams.total(data.totalElements);
return data.content;
});
}
function Delete(id) {
$ngConfirm({
title: 'حذف برنامه',
content: '<span class="text-danger">{{"Are you sure about delete this item?"|translate}}</span>',
scope: $scope,
buttons: {
sayBoo: {
text: 'بله',
btnClass: 'btn-blue',
action: function (scope, button) {
vm.loading = true;
applicationService.deleteApplication(id).then(function () {
vm.applicationTable.reload();
util.toast('success', 'برنامه با موفقیت حذف شد.');
vm.loading = false;
}, function (error) {
if (error.data && error.data.message)
util.toast('error', error.data.message);
else
util.toast('error', 'خطا در حذف برنامه!');
vm.loading = false;
});
return true;
}
},
somethingElse: {
text: 'خیر',
btnClass: 'btn-orange',
action: function (scope, button) {
return true;
}
}
}
});
}
function getCategories() {
return categoryService.getAllCategories();
}
function getOwners() {
return ownerService.getAllOwners();
}
function getUsers() {
return userService.getAllUsers();
}
function getGroup() {
if ($stateParams.groupId)
userGroupService.getUserGroup($stateParams.groupId).then(function (data) {
vm.group = data;
}, function (error) {
util.toast('error', 'گروه مورد نظر یافت نشد!');
});
}
}
})();<file_sep>/*
SQLyog Ultimate v12.09 (64 bit)
MySQL - 5.7.10-log : Database - sbazaar
*********************************************************************
*/
/*!40101 SET NAMES utf8 */;
/*!40101 SET SQL_MODE=''*/;
/*!40014 SET @OLD_UNIQUE_CHECKS=@@UNIQUE_CHECKS, UNIQUE_CHECKS=0 */;
/*!40014 SET @OLD_FOREIGN_KEY_CHECKS=@@FOREIGN_KEY_CHECKS, FOREIGN_KEY_CHECKS=0 */;
/*!40101 SET @OLD_SQL_MODE=@@SQL_MODE, SQL_MODE='NO_AUTO_VALUE_ON_ZERO' */;
/*!40111 SET @OLD_SQL_NOTES=@@SQL_NOTES, SQL_NOTES=0 */;
CREATE DATABASE /*!32312 IF NOT EXISTS*/`sbazaar` /*!40100 DEFAULT CHARACTER SET utf8 */;
/*Table structure for table `access` */
DROP TABLE IF EXISTS `access`;
CREATE TABLE `access` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`code` varchar(50) DEFAULT NULL,
`description` text,
`label` varchar(50) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
/*Table structure for table `access_file` */
DROP TABLE IF EXISTS `access_file`;
CREATE TABLE `access_file` (
`id` bigint(20) unsigned NOT NULL,
`access_id` bigint(20) NOT NULL,
`file_id` bigint(20) NOT NULL,
PRIMARY KEY (`id`),
KEY `foreign_key_access_file_file` (`file_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
/*Table structure for table `advertisement` */
DROP TABLE IF EXISTS `advertisement`;
CREATE TABLE `advertisement` (
`id` BIGINT(20) NOT NULL,
`url` VARCHAR(50) DEFAULT NULL,
`picture_id` BIGINT(20) DEFAULT NULL,
`user_id` VARCHAR(50) DEFAULT NULL,
`fromDate` DATETIME DEFAULT NULL,
`toDate` DATETIME DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
/*Table structure for table `application` */
DROP TABLE IF EXISTS `application`;
CREATE TABLE `application` (
`id` bigint(20) unsigned NOT NULL,
`app_package` varchar(255) DEFAULT NULL,
`fee` int(11) DEFAULT NULL,
`name` varchar(255) DEFAULT NULL,
`category_id` bigint(20) unsigned DEFAULT NULL,
`company_id` bigint(20) unsigned DEFAULT NULL,
`logo_id` bigint(20) unsigned DEFAULT NULL,
`user_id` varchar(50) DEFAULT NULL,
`create_date` datetime DEFAULT NULL,
`last_update` datetime DEFAULT NULL,
`specific_app` binary(1) DEFAULT '0',
`banner_id` bigint(20) unsigned DEFAULT NULL,
`new_app` BINARY(1) DEFAULT '0',
`last_file_id` BIGINT(20) UNSIGNED DEFAULT NULL,
PRIMARY KEY (`id`),
UNIQUE KEY `unque` (`app_package`),
KEY `foreign_key_application_picture` (`logo_id`),
KEY `foreign_key_application_company` (`company_id`),
KEY `foreign_key_application_category` (`category_id`),
KEY `foreign_key_application_picture_2` (`banner_id`),
KEY `foreign_key_application_file` (`last_file_id`),
CONSTRAINT `foreign_key_application_category` FOREIGN KEY (`category_id`) REFERENCES `category` (`id`) ON DELETE NO ACTION ON UPDATE CASCADE,
CONSTRAINT `foreign_key_application_company` FOREIGN KEY (`company_id`) REFERENCES `owner` (`id`) ON DELETE NO ACTION ON UPDATE CASCADE,
CONSTRAINT `foreign_key_application_picture` FOREIGN KEY (`logo_id`) REFERENCES `picture` (`id`) ON DELETE NO ACTION ON UPDATE CASCADE,
CONSTRAINT `foreign_key_application_picture_2` FOREIGN KEY (`banner_id`) REFERENCES `picture` (`id`) ON DELETE NO ACTION ON UPDATE CASCADE
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
/*Table structure for table `authorities` */
DROP TABLE IF EXISTS `authorities`;
CREATE TABLE `authorities` (
`username` varchar(50) NOT NULL,
`authority` varchar(45) NOT NULL,
PRIMARY KEY (`username`,`authority`),
KEY `fk_authoroties_1_idx` (`username`),
CONSTRAINT `fk_authoroties_1` FOREIGN KEY (`username`) REFERENCES `user` (`username`) ON DELETE NO ACTION ON UPDATE NO ACTION
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
/*Table structure for table `category` */
DROP TABLE IF EXISTS `category`;
CREATE TABLE `category` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`name` varchar(255) DEFAULT NULL,
`picture_id` bigint(20) unsigned DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `foreign_key_category_picture` (`picture_id`),
CONSTRAINT `foreign_key_category_picture` FOREIGN KEY (`picture_id`) REFERENCES `picture` (`id`) ON DELETE CASCADE ON UPDATE CASCADE
) ENGINE=InnoDB AUTO_INCREMENT=5 DEFAULT CHARSET=utf8;
/*Table structure for table `comment` */
DROP TABLE IF EXISTS `comment`;
CREATE TABLE `comment` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`content` varchar(255) DEFAULT NULL,
`content_id` bigint(20) unsigned DEFAULT NULL,
`date` datetime DEFAULT NULL,
`user_id` varchar(45) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `foreign_key_comment_contetn` (`content_id`),
CONSTRAINT `foreign_key_comment_contetn` FOREIGN KEY (`content_id`) REFERENCES `content` (`id`) ON DELETE CASCADE ON UPDATE CASCADE
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
/*Table structure for table `owner` */
DROP TABLE IF EXISTS `owner`;
CREATE TABLE `owner` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`name` varchar(255) DEFAULT NULL,
`url` varchar(255) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=5 DEFAULT CHARSET=utf8;
/*Table structure for table `content` */
DROP TABLE IF EXISTS `content`;
CREATE TABLE `content` (
`id` BIGINT(20) UNSIGNED NOT NULL AUTO_INCREMENT,
`description` text,
`seen_count` INT(11) UNSIGNED DEFAULT '0',
`title` VARCHAR(255) DEFAULT NULL,
`type` VARCHAR(255) DEFAULT NULL,
`DTYPE` VARCHAR(45) DEFAULT NULL,
`rating` DOUBLE DEFAULT '0',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=6 DEFAULT CHARSET=utf8;
/*Table structure for table `feedback` */
DROP TABLE IF EXISTS `feedback`;
CREATE TABLE `feedback` (
`id` BIGINT(20) UNSIGNED NOT NULL AUTO_INCREMENT,
`date` DATETIME DEFAULT NULL,
`liked` TINYINT(1) DEFAULT '0',
`disliked` TINYINT(1) DEFAULT '0',
`rate` INT(11) DEFAULT '0',
`content_id` BIGINT(20) UNSIGNED DEFAULT NULL,
`username` VARCHAR(50) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `foreign_key_feedback_content` (`content_id`),
KEY `foreign_key_feedback_user` (`username`),
CONSTRAINT `foreign_key_feedback_content` FOREIGN KEY (`content_id`) REFERENCES `content` (`id`)
ON DELETE CASCADE
ON UPDATE CASCADE,
CONSTRAINT `foreign_key_feedback_user` FOREIGN KEY (`username`) REFERENCES `user` (`username`)
ON DELETE CASCADE
ON UPDATE CASCADE
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
/*Table structure for table `file` */
DROP TABLE IF EXISTS `file`;
CREATE TABLE `file` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`application_id` bigint(20) DEFAULT NULL,
`data` longblob,
`description` text,
`name` varchar(255) DEFAULT NULL,
`registration_date` datetime DEFAULT NULL,
`size` int(11) DEFAULT NULL,
`version` varchar(255) DEFAULT NULL,
PRIMARY KEY (`id`)
)
ENGINE = InnoDB
AUTO_INCREMENT = 23
DEFAULT CHARSET = utf8;
/*Table structure for table `news` */
DROP TABLE IF EXISTS `news`;
CREATE TABLE `news` (
`id` bigint(20) unsigned NOT NULL,
`context` text,
`main_picture_id` bigint(20) DEFAULT NULL,
`date` datetime NOT NULL,
`content_id` varchar(45) DEFAULT NULL,
PRIMARY KEY (`id`),
UNIQUE KEY `id_UNIQUE` (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
/*Table structure for table `picture` */
DROP TABLE IF EXISTS `picture`;
CREATE TABLE `picture` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`content_id` bigint(20) unsigned DEFAULT NULL,
`data` longblob,
`label` varchar(255) DEFAULT NULL,
`type` varchar(255) DEFAULT NULL,
`published` tinyint(1) DEFAULT NULL,
`format` varchar(64) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `foreign_key_picture_content` (`content_id`),
CONSTRAINT `foreign_key_picture_content` FOREIGN KEY (`content_id`) REFERENCES `content` (`id`) ON DELETE CASCADE ON UPDATE CASCADE
)
ENGINE = InnoDB
AUTO_INCREMENT = 27
DEFAULT CHARSET = utf8;
/*Table structure for table `role` */
DROP TABLE IF EXISTS `role`;
CREATE TABLE `role` (
`authority` VARCHAR(50) NOT NULL,
`caption` VARCHAR(50) DEFAULT NULL,
PRIMARY KEY (`authority`)
)
ENGINE = InnoDB
DEFAULT CHARSET = utf8;
/*Table structure for table `transaction` */
DROP TABLE IF EXISTS `transaction`;
CREATE TABLE `transaction` (
`id` BIGINT(20) UNSIGNED NOT NULL AUTO_INCREMENT,
`date` DATETIME DEFAULT NULL,
`amount` INT(11) DEFAULT NULL,
`type` VARCHAR(20) DEFAULT NULL,
`app_id` BIGINT(20) UNSIGNED DEFAULT NULL,
`transaction_id` BIGINT(20) UNSIGNED DEFAULT NULL,
`reference_id` BIGINT(20) UNSIGNED DEFAULT NULL,
`username` VARCHAR(50) DEFAULT NULL,
`comment` VARCHAR(1024) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `transaction_fk1_username` (`username`),
KEY `transaction_fk2_appId` (`app_id`),
CONSTRAINT `transaction_fk1_username` FOREIGN KEY (`username`) REFERENCES `user` (`username`)
ON DELETE NO ACTION
ON UPDATE CASCADE,
CONSTRAINT `transaction_fk2_appId` FOREIGN KEY (`app_id`) REFERENCES `content` (`id`)
ON DELETE NO ACTION
ON UPDATE CASCADE
)
ENGINE = InnoDB
AUTO_INCREMENT = 11
DEFAULT CHARSET = utf8;
/*Table structure for table `user` */
DROP TABLE IF EXISTS `user`;
CREATE TABLE `user` (
`username` VARCHAR(50) NOT NULL,
`password` VARCHAR(60) NOT NULL,
`enabled` TINYINT(4) DEFAULT '1',
`name` VARCHAR(45) DEFAULT NULL,
`family` VARCHAR(45) DEFAULT NULL,
`address` VARCHAR(45) DEFAULT NULL,
`mobile_phone` VARCHAR(13) DEFAULT NULL,
`mobile_phone_two` VARCHAR(13) DEFAULT NULL,
`mobile_phone_three` VARCHAR(13) DEFAULT NULL,
`phone` VARCHAR(14) DEFAULT NULL,
`postal_code` VARCHAR(45) DEFAULT NULL,
`email` VARCHAR(45) DEFAULT NULL,
`profile_id` BIGINT(20) UNSIGNED DEFAULT NULL,
`credit` INT(11) DEFAULT '0',
`score` INT(11) DEFAULT '0',
`imei` VARCHAR(40) DEFAULT NULL,
PRIMARY KEY (`username`),
KEY `user_fk1_profile_pic` (`profile_id`),
CONSTRAINT `user_fk1_profile_pic` FOREIGN KEY (`profile_id`) REFERENCES `picture` (`id`)
ON DELETE NO ACTION
ON UPDATE CASCADE
)
ENGINE = InnoDB
DEFAULT CHARSET = utf8;
/*Table structure for table `user_app` */
DROP TABLE IF EXISTS `user_app`;
CREATE TABLE `user_app` (
`id` BIGINT(20) UNSIGNED NOT NULL AUTO_INCREMENT,
`username` VARCHAR(50) DEFAULT NULL,
`app_id` BIGINT(20) UNSIGNED DEFAULT NULL,
`install_date` DATETIME DEFAULT NULL,
`uninstall_date` DATETIME DEFAULT NULL,
`phone_number` VARCHAR(14) DEFAULT NULL,
`current_version` VARCHAR(255) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `user_app_fk1_username` (`username`),
KEY `user_app_fk2_app_id` (`app_id`),
CONSTRAINT `user_app_fk1_username` FOREIGN KEY (`username`) REFERENCES `user` (`username`)
ON DELETE CASCADE
ON UPDATE CASCADE,
CONSTRAINT `user_app_fk2_app_id` FOREIGN KEY (`app_id`) REFERENCES `content` (`id`)
ON DELETE CASCADE
ON UPDATE CASCADE
)
ENGINE = InnoDB
AUTO_INCREMENT = 2
DEFAULT CHARSET = utf8;
/*!40101 SET SQL_MODE=@OLD_SQL_MODE */;
/*!40014 SET FOREIGN_KEY_CHECKS=@OLD_FOREIGN_KEY_CHECKS */;
/*!40014 SET UNIQUE_CHECKS=@OLD_UNIQUE_CHECKS */;
/*!40111 SET SQL_NOTES=@OLD_SQL_NOTES */;
<file_sep>package ir.saafta.model;
import ir.saafta.dto.ApplicationStatus;
import ir.saafta.dto.SessionStatus;
import org.springframework.beans.factory.annotation.Required;
import org.springframework.beans.factory.annotation.Value;
import javax.persistence.*;
import javax.validation.constraints.NotNull;
import javax.ws.rs.DefaultValue;
import java.util.*;
/**
* Created by reza on 11/12/16.
*/
@Entity
public class Application extends Content {
@OneToMany(mappedBy = "applicationId")
private Collection<File> files;
@NotNull
private String appPackage;
@DefaultValue("0")
private Integer fee;
private Boolean inAppPayment;
@NotNull
private String name;
private Date createDate;
private Date lastUpdate;
private Boolean specificApp;
private Boolean newApp;
private Boolean recentlyUpdated;
@Value("${suggestedApp:false}")
private Boolean suggestedApp;
private Integer suggestionPriority;
/*@ManyToOne
@JoinColumn(name = "suggestionPriority")
private SuggestionPriority suggestionPriority;*/
@ManyToOne
@JoinColumn(name = "categoryId")
private Category category;
@ManyToOne
@JoinColumn(name = "ownerId")
private Owner owner;
@OneToOne
@JoinColumn(name = "logoId")
private Picture logo;
@OneToOne
@JoinColumn(name = "bannerId")
private Picture banner;
@ManyToOne
@JoinColumn(name = "userId")
private User user;
private Long lastFileId;
private Integer installationCount;
@Transient
private Double userRating;
@Transient
private Boolean userBookmarked;
@Transient
private Boolean isMine;
private String originalName;
@Enumerated(EnumType.ORDINAL)
private ApplicationStatus status;
public Boolean checkIncomplete() {
return this.logo == null || this.getPictures() == null || this.getPictures().size() == 0;
}
public String getAppPackage() {
return appPackage;
}
public void setAppPackage(String appPackage) {
this.appPackage = appPackage;
}
public Integer getFee() {
return fee;
}
public void setFee(Integer fee) {
this.fee = fee;
}
public Boolean getInAppPayment() {
return inAppPayment;
}
public void setInAppPayment(Boolean inAppPayment) {
this.inAppPayment = inAppPayment;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
super.setTitle(name);
}
public Category getCategory() {
return category;
}
@Required
public void setCategory(Category category) {
this.category = category;
}
public Picture getLogo() {
return logo;
}
public void setLogo(Picture logo) {
this.logo = logo;
}
public User getUser() {
return user;
}
public void setUser(User user) {
this.user = user;
}
public Owner getOwner() {
return owner;
}
public void setOwner(Owner owner) {
this.owner = owner;
}
public Date getCreateDate() {
return createDate;
}
public void setCreateDate(Date createDate) {
this.createDate = createDate;
}
public Date getLastUpdate() {
return lastUpdate;
}
public void setLastUpdate(Date lastUpdate) {
this.lastUpdate = lastUpdate;
}
public Collection<File> getFiles() {
return files;
}
public void setFiles(Collection<File> files) {
this.files = files;
}
@Override
public Collection<Picture> getPictures() {
if (super.getPictures() == null || super.getPictures().size() == 0)
return null;
ArrayList<Picture> sPictures = new ArrayList<>(super.getPictures());
sPictures.removeIf(pic -> !pic.getType().matches("app"));
return sPictures;
}
public Boolean getSpecificApp() {
return specificApp;
}
public void setSpecificApp(Boolean specificApp) {
this.specificApp = specificApp;
}
public Picture getBanner() {
return banner;
}
public void setBanner(Picture banner) {
this.banner = banner;
}
public Boolean getNewApp() {
return newApp;
}
public void setNewApp(Boolean newApp) {
this.newApp = newApp;
}
public Long getLastFileId() {
return lastFileId;
}
public void setLastFileId(Long lastFileId) {
this.lastFileId = lastFileId;
}
@Transient
public File getLastFile() {
if (this.lastFileId != null) {
for (File file : this.files) {
if (file.getId().equals(this.lastFileId))
return file;
}
return null;
} else {
return calculateLastFile(null);
}
/*List<File> files = (List<File>) this.files;
if (files == null || files.size() == 0)
return null;
files.sort(Comparator.comparing(File::getRegistrationDate).reversed());
if (files.size() > 0)
return files.get(0);
return null;*/
}
@Transient
public File calculateLastFile(Long removedFileId) {
List<File> files = (List<File>) this.files;
if (files == null || files.size() == 0)
return null;
files.sort(Comparator.comparing(File::getRegistrationDate).reversed());
for (File file : files) {
if (!file.getId().equals(removedFileId))
return file;
}
return null;
}
public Boolean getRecentlyUpdated() {
return recentlyUpdated;
}
public void setRecentlyUpdated(Boolean recentlyUpdated) {
this.recentlyUpdated = recentlyUpdated;
}
public Integer getInstallationCount() {
return installationCount;
}
public void setInstallationCount(Integer installationCount) {
this.installationCount = installationCount;
}
public Boolean getSuggestedApp() {
return suggestedApp;
}
public void setSuggestedApp(Boolean suggestedApp) {
this.suggestedApp = suggestedApp;
}
public Integer getSuggestionPriority() {
return suggestionPriority;
}
public void setSuggestionPriority(Integer suggestionPriority) {
this.suggestionPriority = suggestionPriority;
}
public void decreaseSuggestionPriority() {
if (this.suggestionPriority != null)
this.suggestionPriority++;
}
@Transient
public String getInstallUrl() {
return "download/" + super.getId() + "/" + this.appPackage + ".apk";
}
@Transient
public Double getUserRating() {
return userRating;
}
public void setUserRating(Double userRating) {
this.userRating = userRating;
}
@Transient
public Boolean getUserBookmarked() {
return userBookmarked;
}
public void setUserBookmarked(Boolean userBookmarked) {
this.userBookmarked = userBookmarked;
}
@Transient
public boolean isMyApp(User user) {
return user != null && this.user != null && this.user.equals(user);
}
@Transient
public Boolean getMine() {
return isMine;
}
public void setMine(Boolean mine) {
isMine = mine;
}
public String getOriginalName() {
return originalName;
}
public void setOriginalName(String originalName) {
this.originalName = originalName;
}
public ApplicationStatus getStatus() {
return status;
}
public void setStatus(ApplicationStatus status) {
this.status = status;
}
}
<file_sep>package ir.saafta.repo;
import ir.saafta.model.User;
import ir.saafta.model.UserGroup;
import org.springframework.data.jpa.repository.JpaSpecificationExecutor;
import org.springframework.data.repository.PagingAndSortingRepository;
/**
* Created by reza on 11/12/16.
*/
public interface UserRepository extends PagingAndSortingRepository<User, String>, JpaSpecificationExecutor<User> {
Iterable<User> findAllByUserGroupsIsNotContaining(UserGroup userGroup);
User findByEmail(String email);
User findByMobilePhone(String mobilePhone);
User findByEmailAndUsernameIsNot(String email, String username);
User findByMobilePhoneAndUsernameIsNot(String mobilePhone, String username);
}
<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('CreateUpdateNewsController', CreateUpdateNewsController);
/** @ngInject */
function CreateUpdateNewsController($log, $timeout, newsService, $stateParams, toastr, $state, host) {
var vm = this;
vm.title = 'CreateUpdateUserController';
vm.host = host;
vm.method = $stateParams.method;
vm.news = {
id: $stateParams.id
};
vm.save = save;
vm.reset = reset;
vm.mainPicture = mainPicture;
vm.date = new Date();
activate();
////////////////
function activate() {
$log.debug('News module activated');
getNews();
}
function save() {
vm.loading = true;
switch (vm.method) {
case 'create':
vm.news.seenCount = 0;
newsService.createNews(vm.news)
.then(function (response) {
console.log(response);
$timeout(function () {
$state.go('root.createUpdateNews', {method: 'update', id: response.data.id});
vm.loading = false;
}, 3000);
toastr.success('خبر با موفقیت افزوده شد.');
}, function () {
toastr.error('خطا در ایجاد خبر!');
vm.loading = false;
});
break;
case 'update':
newsService.updateNews(vm.news)
.then(function (data) {
vm.news = data;
toastr.success('خبر با موفقیت ویرایش شد.');
vm.loading = false;
}, function () {
toastr.error('خطا در ویرایش خبر!');
vm.loading = false;
});
break;
default:
vm.loading = false;
}
}
function mainPicture() {
vm.loading = true;
newsService.mainPicture(vm.news.id, vm.cropper.croppedImage).then(
function (data) {
vm.news = data;
vm.date = new Date();
vm.cropper = null;
vm.loading = false;
toastr.success('تصویر خبر با موفقیت بهروزرسانی شد.');
}
);
}
function reset() {
switch (vm.method) {
case 'create':
vm.news = {};
break;
case 'update':
getNews();
}
}
function getNews() {
if (vm.news.id) {
vm.loading = true;
newsService.getNews(vm.news.id).then(function (data) {
vm.loading = false;
vm.news = data;
}, function () {
vm.loading = false;
});
}
}
}
})();<file_sep>package ir.saafta.web;
import ir.saafta.exception.HttpUnauthorizedException;
import ir.saafta.model.Application;
import ir.saafta.model.User;
import ir.saafta.repo.ApplicationRepository;
import ir.saafta.repo.CategoryRepository;
import ir.saafta.repo.OwnerRepository;
import ir.saafta.service.UserDetailManager;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.access.prepost.PreAuthorize;
import org.springframework.security.core.Authentication;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
/**
* Created by reza on 12/3/16.
*/
@RestController
@RequestMapping("/dashboard")
public class DashboardController {
private final String simurghPackage = "ir.simorghmarket.simorgh";
private ApplicationRepository applicationRepository;
private CategoryRepository categoryRepository;
private OwnerRepository ownerRepository;
private UserDetailManager userDetailManager;
@Autowired
public DashboardController(ApplicationRepository applicationRepository, UserDetailManager userDetailManager,
CategoryRepository categoryRepository, OwnerRepository ownerRepository) {
this.applicationRepository = applicationRepository;
this.categoryRepository = categoryRepository;
this.ownerRepository = ownerRepository;
this.userDetailManager = userDetailManager;
}
@PreAuthorize("hasAuthority('admin')")
@RequestMapping(method = RequestMethod.GET)
public Map<String, Object> dashboard() {
Map result = new HashMap<String, Object>();
int minus = 0;
minus += applicationRepository.countApplicationsByAppPackage(simurghPackage);
result.put("applications", applicationRepository.count() - minus);
result.put("users", userDetailManager.count());
result.put("categories", categoryRepository.count());
result.put("owners", ownerRepository.count());
return result;
}
@PreAuthorize("isAuthenticated()")
@RequestMapping(value = "/me", method = RequestMethod.GET)
public Map<String, Object> myDashboard(Authentication authentication) {
if(authentication == null){
throw new HttpUnauthorizedException();
}
User user = (User) userDetailManager.loadUserByUsername(authentication.getName());
Map result = new HashMap<String, Object>();
result.put("applications", applicationRepository.findAllByUser(user).size());
result.put("credit", user.getCredit() == null ? 0 : user.getCredit());
result.put("averageRating", getAverageRating(applicationRepository.findAllByUser(user)));
return result;
}
Double getAverageRating(Collection<Application> apps) {
if(apps.isEmpty())
return 0.0;
Double result = 0.0;
for (Application app : apps) {
app.setRating(app.getRating() == null ? 0 : app.getRating());
result += app.getRating();
}
return result/apps.size();
}
}
<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('MainController', MainController);
/** @ngInject */
function MainController($document, $window) {
var vm = this;
var firstLogin = $document[0].cookie.replace(
/(?:(?:^|.*;\s*)firstLogin\s*\=\s*([^;]*).*$)|^.*$/, '$1'
);
activate();
function activate() {
if (!firstLogin) {
$document[0].cookie = 'firstLogin=true';
}
vm.isIE = detectIE();
}
function detectIE() {
var ua = window.navigator.userAgent;
// Test values; Uncomment to check result …
// IE 10
// ua = 'Mozilla/5.0 (compatible; MSIE 10.0; Windows NT 6.2; Trident/6.0)';
// IE 11
// ua = 'Mozilla/5.0 (Windows NT 6.3; Trident/7.0; rv:11.0) like Gecko';
// Edge 12 (Spartan)
// ua = 'Mozilla/5.0 (Windows NT 10.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/39.0.2171.71 Safari/537.36 Edge/12.0';
// Edge 13
// ua = 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/46.0.2486.0 Safari/537.36 Edge/13.10586';
var msie = ua.indexOf('MSIE ');
if (msie > 0) {
// IE 10 or older => return version number
return parseInt(ua.substring(msie + 5, ua.indexOf('.', msie)), 10);
}
var trident = ua.indexOf('Trident/');
if (trident > 0) {
// IE 11 => return version number
var rv = ua.indexOf('rv:');
return parseInt(ua.substring(rv + 3, ua.indexOf('.', rv)), 10);
}
var edge = ua.indexOf('Edge/');
if (edge > 0) {
// Edge (IE 12+) => return version number
return parseInt(ua.substring(edge + 5, ua.indexOf('.', edge)), 10);
}
// other browser
return false;
}
}
})();
<file_sep>(function () {
'use strict';
angular
.module('app')
.factory('applicationService', applicationService);
/** @ngInject */
function applicationService($log, $http, host) {
var base = host + 'application/';
return {
getApplication: getApplication,
getSimurgh: getSimurgh
};
////////////////
function getApplication(id) {
return $http.get(base + id);
}
function getSimurgh(params) {
var url = base + 'simorgh';
return $http.get(url, {params: {type: 'nonLuncher'}})
.then(successCallback);
}
function successCallback(response) {
return response.data;
}
}
})();<file_sep>(function () {
'use strict';
angular
.module('auth')
.controller('RegisterController', RegisterController);
/** @ngInject */
function RegisterController($log) {
var vm = this;
vm.title = 'RegisterController';
activate();
////////////////
function activate() {
$log.info('register module ativated.');
}
}
})();
<file_sep>package ir.saafta.component;
import ir.saafta.model.SpringSession;
import ir.saafta.model.User;
import ir.saafta.repo.SpringSessionRepository;
import ir.saafta.repo.UserRepository;
import org.jasypt.encryption.pbe.StandardPBEStringEncryptor;
import org.jasypt.properties.EncryptableProperties;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.security.core.session.SessionRegistry;
import org.springframework.stereotype.Component;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.FileInputStream;
import java.util.Arrays;
import java.util.Properties;
@Component
public class InitializingSessionRegistry implements InitializingBean {
private static final Logger log = LoggerFactory.getLogger(InitializingSessionRegistry.class);
@Autowired
private SpringSessionRepository springSessionRepository;
@Autowired
private UserRepository userRepository;
@Autowired
@Qualifier("sessionRegistry")
private SessionRegistry sessionRegistry;
@Override
public void afterPropertiesSet() throws Exception {
log.info("Initializing session registry " + springSessionRepository.findAllByPrincipalNameIsNotNull().size());
for (SpringSession springSession : springSessionRepository.findAllByPrincipalNameIsNotNull()) {
if (sessionRegistry.getSessionInformation(springSession.getSessionId()) == null) {
log.info(springSession.getSessionId() + " is not registered!");
User user = userRepository.findOne(springSession.getPrincipalName());
sessionRegistry.registerNewSession(springSession.getSessionId(), user);
}
}
log.info(sessionRegistry.getAllPrincipals().toString());
}
}
<file_sep>package ir.saafta.service;
import ir.saafta.model.UserGroup;
import ir.saafta.repo.UserGroupRepository;
import org.apache.xmlbeans.impl.xb.xsdschema.Public;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
/**
* Created by sft940026 on 14/09/2017.
*/
@Component
public class UserGroupService {
private final UserGroupRepository userGroupRepository;
@Autowired
public UserGroupService(UserGroupRepository userGroupRepository) {
this.userGroupRepository = userGroupRepository;
}
public UserGroup getPublicGroup() {
return userGroupRepository.findOne(1L);
}
public UserGroup getById(Long id) {
return userGroupRepository.findOne(id);
}
}
<file_sep>/**
* Created by reza on 11/14/16.
*/
(function () {
'use strict';
angular
.module('app')
.config(routeConfig);
/** @ngInject */
function routeConfig($stateProvider) {
$stateProvider
.state('root.event', {
controller: 'EventController',
controllerAs: 'vm',
data: {
roles: [
"admin"
]
},
resolve: {},
templateUrl: 'app/components/event/list-event.html',
url: '/event?category'
})
;
}
})();
<file_sep>/**
* Created by reza on 11/14/16.
*/
(function () {
'use strict';
angular
.module('app')
.config(routeConfig);
/** @ngInject */
function routeConfig($stateProvider) {
$stateProvider
.state('root.news', {
controller: 'NewsController',
controllerAs: 'vm',
data: {
roles: [
"admin"
]
},
resolve: {},
templateUrl: 'app/components/news/list-news.html',
url: '/news'
})
.state('root.createUpdateNews', {
controller: 'CreateUpdateNewsController',
controllerAs: 'vm',
data: {
roles: [
"admin"
]
},
resolve: {},
templateUrl: 'app/components/news/create-update-news.html',
url: '/news/:method?id'
})
;
}
})();
<file_sep>(function () {
'use strict';
/**
* @ngdoc service
* @name app.service:sidebarService
* @description
* # sidebarService
*/
angular
.module('app')
.service('sidebarService', sidebarService);
/** @ngInject */
function sidebarService($http) {
/* jshint validthis: true */
this.getSidebar = getSidebar;
//////////
function getSidebar() {
return $http.get('data/sidebar.json').then(successCallback)
}
function successCallback(response) {
return response.data;
}
}
})();<file_sep>package ir.saafta.model;
import javax.persistence.*;
import java.util.Date;
/**
* Created by reza on 11/14/16.
*/
@Entity
@Inheritance(strategy = InheritanceType.JOINED)
public class Feedback {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private Date date;
private Boolean liked;
private Boolean disliked;
private Double rate;
private Long contentId;
@ManyToOne
@JoinColumn(name = "username")
private User user;
public Feedback() {
}
public Feedback(Date date, Boolean like, Boolean disliked, Double rate, Long contentId, User user) {
this.date = date;
this.liked = like;
this.disliked = disliked;
this.rate = rate;
this.contentId = contentId;
this.user = user;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public Date getDate() {
return date;
}
public void setDate(Date date) {
this.date = date;
}
public Boolean getLiked() {
return liked;
}
public void setLiked(Boolean liked) {
this.liked = liked;
}
public Boolean getDisliked() {
return disliked;
}
public void setDisliked(Boolean disliked) {
this.disliked = disliked;
}
public Double getRate() {
return rate;
}
public void setRate(Double rate) {
this.rate = rate;
}
public Long getContentId() {
return contentId;
}
public void setContentId(Long contentId) {
this.contentId = contentId;
}
public User getUser() {
return user;
}
public void setUser(User user) {
this.user = user;
}
}
<file_sep>package ir.saafta.conf;
import ir.saafta.dto.SessionStatus;
import ir.saafta.model.Session;
import ir.saafta.repo.MySessionRepository;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.session.SessionInformation;
import org.springframework.security.core.session.SessionRegistry;
import org.springframework.security.web.authentication.session.ConcurrentSessionControlAuthenticationStrategy;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.util.List;
public class CustomSessionAuthenticationStrategy extends ConcurrentSessionControlAuthenticationStrategy {
private final SessionRegistry sessionRegistry;
private final MySessionRepository sessionRepository;
private final Logger logger = LoggerFactory.getLogger(this.getClass());
/**
* @param sessionRegistry the session registry which should be updated when the
* authenticated session is changed.
*/
public CustomSessionAuthenticationStrategy(SessionRegistry sessionRegistry) {
super(sessionRegistry);
this.sessionRegistry = sessionRegistry;
this.sessionRepository = null;
}
public CustomSessionAuthenticationStrategy(SessionRegistry sessionRegistry, MySessionRepository sessionRepository) {
super(sessionRegistry);
this.sessionRegistry = sessionRegistry;
this.sessionRepository = sessionRepository;
}
@Override
public void onAuthentication(Authentication authentication,
HttpServletRequest request, HttpServletResponse response) {
logger.info("onAuthentication `" + authentication.getName() + "`\tsession: " + request.getSession().getId());
final List<SessionInformation> sessions = sessionRegistry.getAllSessions(
authentication.getPrincipal(), false);
int sessionCount = sessions.size();
int allowedSessions = getMaximumSessionsForThisUser(authentication);
if (sessionCount < allowedSessions) {
// They haven't got too many login sessions running at present
return;
}
if (allowedSessions == -1) {
// We permit unlimited logins
return;
}
if (sessionCount == allowedSessions) {
HttpSession session = request.getSession(false);
if (session != null) {
// Only permit it though if this request is associated with one of the
// already registered sessions
for (SessionInformation si : sessions) {
logger.info("\t" + si.getSessionId());
Session storedSession = sessionRepository.findOne(si.getSessionId());
logger.info("\t" + storedSession.getDeviceInfo() + " vs " + request.getHeader("User-Agent"));
logger.info("\t\t" + storedSession.getDeviceInfo().compareTo(request.getHeader("User-Agent")));
if (storedSession.getDeviceInfo().compareTo(request.getHeader("User-Agent")) == 0/* && storedSession.getDeviceInfo().startsWith("_app")*/) {
si.expireNow();
storedSession.setStatus(SessionStatus.TERMINATED);
sessionRepository.save(storedSession);
return;
}
if (si.getSessionId().equals(session.getId())) {
return;
}
}
}
// If the session is null, a new one will be created by the parent class,
// exceeding the allowed number
}
allowableSessionsExceeded(sessions, allowedSessions, sessionRegistry);
}
}
<file_sep>/**
* Created by reza on 11/14/16.
*/
(function () {
'use strict';
angular
.module('app')
.config(routeConfig);
/** @ngInject */
function routeConfig($stateProvider) {
$stateProvider
.state('root.category', {
controller: 'CategoryController',
controllerAs: 'vm',
data: {
roles: [
"admin"
]
},
resolve: {},
templateUrl: 'app/components/category/list-category.html',
url: '/category'
})
.state('root.createUpdateCategory', {
controller: 'CreateUpdateCategoryController',
controllerAs: 'vm',
data: {
roles: [
"admin"
]
},
resolve: {},
templateUrl: 'app/components/category/create-update-category.html',
url: '/category/:method?id'
})
;
}
})();
<file_sep>(function () {
'use strict';
angular
.module('auth')
.controller('LoginController', LoginController);
/** @ngInject */
function LoginController($log, $http, $state, host, toastr) {
var vm = this;
vm.title = 'LoginController';
vm.host = host;
vm.login = login;
activate();
////////////////
function activate() {
$log.info('login module activated.');
}
function login() {
var url = host + 'login';
$http({
method: 'POST',
url: url,
withCredentials: true,
data: "username=" + vm.username + '&password=' + <PASSWORD>,
headers: {'Content-Type': 'application/x-www-form-urlencoded'}
}).then(function () {
$log.debug('login successful');
$state.go('root.home');
}, function () {
toastr.error('نام کاربری یا کلمه عبور نادرست است.')
});
}
}
})();
<file_sep>(function () {
'use strict';
angular
.module('app')
.directive('backButton', backButton);
/** @ngInject */
function backButton() {
var directive = {
link: link,
replace: true,
restrict: 'E',
templateUrl: 'app/directives/back-button/back-button.html'
};
return directive;
function link(scope, element) {
element.bind('click', goBack);
function goBack() {
history.back();
scope.$apply();
}
}
}
})();
<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('CreateUpdateApplicationController', CreateUpdateApplicationController);
/** @ngInject */
function CreateUpdateApplicationController($log, $q, $state, applicationService, categoryService, ownerService, $scope,
host, $stateParams, userService, $ngConfirm, $uibModal, util, $window,
accessService, userGroupService) {
var vm = this;
var applicationTmp = null;
vm.host = host;
vm.croppedDataUrl = '';
vm.cropper = {};
vm.cropper.sourceImage = null;
vm.cropper.croppedImage = null;
vm.cropperWidth = $window.innerWidth * 0.4;
vm.bounds = {
left: 0,
right: vm.cropperWidth * 0.5,
top: vm.cropperWidth * 0.4,
bottom: 0
};
vm.bannerBounds = {
left: 0,
right: 20,
top: 20,
bottom: 0
};
vm.resultImage = '';
vm.title = 'CreateUpdateUserController';
vm.method = $stateParams.method;
vm.application = {
id: $stateParams.id,
seenCount: 0,
newApp: false,
specificApp: false,
launcher: false,
nonLauncher: false,
rating: 0
};
vm.file = {};
vm.tmpData = 'nothing';
vm.errors = [];
vm.error = {};
vm.fileLoading = true;
vm.statuses = [
{'id': 'DEACTIVATED', 'title': 'غیرفعال'},
{'id': 'PUBLIC', 'title': 'عمومی'},
{'id': 'LAUNCHER', 'title': 'پوسته امن'},
{'id': 'NON_LAUNCHER', 'title': 'پوسته عادی'}
];
vm.save = save;
vm.reset = reset;
vm.uploadLogo = uploadLogo;
vm.uploadBanner = uploadBanner;
vm.uploadPic = uploadPic;
vm.deletePic = deletePic;
vm.addFileModal = addFileModal;
vm.deleteFile = deleteFile;
vm.initiateSuggestion = initiateSuggestion;
vm.getRatioDiff = getRatioDiff;
vm.deleteBanner = deleteBanner;
vm.setActiveVersion = setActiveVersion;
vm.addGroup = addGroup;
vm.removeGroup = removeGroup;
activate();
////////////////
$scope.$watch(angular.bind(this, function () {
return this.cropper.croppedImage;
}), function (newVal) {
// console.log('cropper changed to ', newVal);
if (document.getElementById('source-image')) {
var w = document.getElementById('source-image').clientWidth;
var h = document.getElementById('source-image').clientHeight;
console.log(w, h);
}
});
function activate() {
$log.debug('Application module activated', $stateParams.id);
vm.simorgh = $stateParams.id === 'simorgh';
if (vm.simorgh) {
$log.debug('This is is simorgh');
if (vm.method === 'create') {
vm.application.appPackage = 'ir.simorghmarket.simorgh';
vm.application.id = null;
vm.application.fee = 0;
}
} else {
if (vm.method === 'create') {
vm.application.fee = 0;
}
}
vm.loading = true;
getUserGroups();
$q.all(getCategories(), getOwners(), getUsers(), getAccesses()).then(getApplication);
}
function save() {
vm.errors = [];
if (!vm.application.name)
vm.errors.push('APP.MODULES.APPLICATION.ERRORS.NAME');
if (!vm.application.appPackage || !util.isEnglish(vm.application.appPackage))
vm.errors.push('APP.MODULES.APPLICATION.ERRORS.APP_PACKAGE');
if ((isNaN(parseInt(vm.application.fee)) || parseInt(vm.application.fee) < 0) && !vm.simorgh)
vm.errors.push('APP.MODULES.APPLICATION.ERRORS.FEE');
console.log(vm.application.category, !vm.application.category);
if ((!vm.application.category || vm.application.category.id === 0) && !vm.simorgh)
vm.errors.push('APP.MODULES.APPLICATION.ERRORS.CATEGORY');
if (vm.errors.length)
return;
vm.loading = true;
switch (vm.method) {
case 'create':
if ($stateParams.type)
vm.application.launcher = $stateParams.type === 'launcher';
applicationService.createApplication(vm.application)
.then(function (data) {
util.toast('success', 'برنامه با موفقیت ایجاد شد. میتوانید برای تکیمل آن، در همین صفحه نسبت به افزودن تصاویر و نسخههای آن اقدام نمایید.');
var id = vm.simorgh ? 'simorgh' : data.id;
vm.error = {};
$state.go('root.createUpdateApplication', {method: 'update', id: id});
vm.loading = false;
}, errorCallback);
break;
case 'update':
applicationService.updateApplication(vm.application)
.then(function (data) {
util.toast('success', 'برنامه با موفقیت بروزرسانی شد.');
vm.error = {};
vm.name = data.name;
vm.loading = false;
}, errorCallback);
break;
default:
vm.loading = false;
}
function errorCallback(error) {
console.error(error);
if(error.data.message === "Application package should be unique") {
vm.error['appPackage'] = true;
}
util.toast('error', error.data.message);
vm.loading = false;
}
}
function reset() {
vm.errors = [];
switch (vm.method) {
case 'create':
vm.application = {
seenCount: 0,
newApp: false,
specificApp: false,
rating: 0
};
break;
case 'update':
loadTmp();
}
}
function getApplication() {
vm.loading = true;
if (vm.simorgh) {
return applicationService.getSimurgh($stateParams.type).then(
function (data) {
vm.application = data;
applicationTmp = angular.copy(data);
vm.name = data.name;
vm.loading = false;
getActiveVersion();
}, function (error) {
/*console.log('get simorgh');
vm.method = 'create';
vm.application = {
id: null,
seenCount: 0,
rating:0,
appPackage: "ir.simorghmarket.simorgh"
};*/
vm.loading = false;
$state.go('root.createUpdateApplication', {method: 'create', id: 'simorgh'});
});
} else if (vm.application.id) {
return applicationService.getApplication(vm.application.id).then(function (data) {
vm.application = data;
applicationTmp = angular.copy(data);
vm.name = data.name;
vm.loading = false;
getActiveVersion();
});
} else {
vm.loading = false;
}
}
function loadTmp() {
vm.application = angular.copy(applicationTmp);
}
function getCategories() {
return categoryService.getAllCategories().then(function (data) {
vm.categories = data;
})
}
function getOwners() {
return ownerService.getAllOwners().then(function (data) {
vm.owners = data;
})
}
function getUsers() {
return userService.getAllUsers().then(function (data) {
vm.users = data;
})
}
function getUserGroups() {
userGroupService.getAllUserGroups().then(function (data) {
vm.groups = data;
})
}
function getAccesses() {
accessService.getAllAccesses().then(
function (data) {
vm.accesses = data;
}
)
}
function uploadLogo(dataUrl) {
vm.loading = true;
applicationService.updateLogo(vm.application.id, dataUrl)
.then(
function (response) {
vm.application.logo = response;
vm.appFile = null;
vm.tmpData = new Date();
util.toast('success', 'نشان با موفقیت به روزرسانی شد.');
vm.cropper = {};
vm.cropper.sourceImage = null;
vm.cropper.croppedImage = null;
vm.loading = false;
},
function (response) {
if (response.status > 0) {
vm.errorMsg = response.status + ': ' + response.data;
}
util.toast('error', 'خطا در بارگذاری تصویر!');
vm.loading = false;
},
function (evt) {
vm.progress = parseInt(100.0 * evt.loaded / evt.total);
vm.loading = false;
});
}
function uploadBanner(dataUrl) {
vm.loading = true;
applicationService.updateBanner(vm.application.id, dataUrl)
.then(
function (response) {
vm.application.banner = response;
vm.appFile = null;
vm.tmpData = new Date();
util.toast('success', 'بنر با موفقیت به روزرسانی شد.');
vm.cropperBanner = {};
vm.cropperBanner.sourceImage = null;
vm.cropperBanner.croppedImage = null;
vm.loading = false;
},
function (response) {
if (response.status > 0) {
vm.errorMsg = response.status + ': ' + response.data;
}
util.toast('error', 'خطا در بارگذاری بنر!');
vm.loading = false;
},
function (evt) {
vm.progress = parseInt(100.0 * evt.loaded / evt.total);
vm.loading = false;
});
}
function uploadPic(dataUrl) {
vm.loading = true;
applicationService.addPic(vm.application.id, dataUrl, vm.picLabel)
.then(
function (response) {
vm.application.pictures = response;
vm.picLabel = '';
util.toast('success', 'تصویر با موفقیت اضافه شد.');
vm.picCropper = {};
vm.picCropper.sourceImage = null;
vm.picCropper.croppedImage = null;
vm.loading = false;
},
function (response) {
if (response.status > 0) {
vm.errorMsg = response.status + ': ' + response.data;
}
util.toast('error', 'خطا در بارگذاری تصویر!');
vm.loading = false;
},
function (evt) {
vm.progress = parseInt(100.0 * evt.loaded / evt.total);
vm.loading = false;
});
}
function deletePic(id) {
vm.loading = true;
applicationService.deletePic(vm.application.id, id)
.then(
function (response) {
vm.application.pictures = response;
util.toast('success', 'تصویر با موفقیت حذف شد.');
vm.picCropper = {};
vm.picCropper.sourceImage = null;
vm.picCropper.croppedImage = null;
vm.loading = false;
},
function (response) {
if (response.status > 0) {
vm.errorMsg = response.status + ': ' + response.data;
}
util.toast('error', 'خطا در حذف تصویر!');
vm.loading = false;
},
function (evt) {
vm.progress = parseInt(100.0 * evt.loaded / evt.total);
vm.loading = false;
});
}
function addFileModal(file) {
file = file || {
description: '',
version: ''
};
var modalInstance = $uibModal.open({
animation: true,
templateUrl: 'app/components/application/file/create-update-file.html',
controller: CreateUpdateFileController,
controllerAs: 'fmodal',
size: 'lg',
backdrop: 'static',
resolve: {
file: function () {
return file;
},
application: function () {
return vm.application;
}
}
});
modalInstance.result.then(function () {
applicationService.getFiles(vm.application.id).then(function (data) {
vm.application.files = data;
});
});
function CreateUpdateFileController(host, file, application, $timeout,
$uibModalInstance, Upload) {
var fmodal = this;
fmodal.application = application;
fmodal.accesses = vm.accesses;
fmodal.file = file;
fmodal.method = file.id ? 'PUT' : 'POST';
var id = file.id || application.id;
fmodal.uploadFile = uploadFile;
fmodal.cancel = function () {
loadTmp();
$uibModalInstance.dismiss();
};
function uploadFile(dataFile) {
fmodal.loading = true;
var accessesStr = '';
if (fmodal.file.accesses) {
for (var i = 0; i < fmodal.file.accesses.length; i++) {
accessesStr += fmodal.file.accesses[i].id;
if (i < fmodal.file.accesses.length) {
accessesStr += ',';
}
}
}
fmodal.upload =
Upload.upload({
url: host + 'application/' + id + '/file',
method: fmodal.method,
data: {
data: dataFile,
description: fmodal.file.description,
version: fmodal.file.version,
minimumAndroidVersion: fmodal.file.minimumAndroidVersion,
accessesStr: accessesStr
}
});
vm.loading = true;
fmodal.upload.then(function (response) {
$timeout(function () {
console.log('file added');
$uibModalInstance.close();
fmodal.result = response.data;
vm.loading = false;
fmodal.loading = false;
});
util.toast('success', 'APP.MESSAGES.SUCCESS');
}, function (response) {
if (response && response.data) {
fmodal.errorMsg = response.status + ': ' + response.data;
util.toast('error', response.data.message);
} else {
util.toast('erorr', 'APP.MESSAGES.FAIL');
}
vm.loading = false;
fmodal.loading = false;
}, function (evt) {
// Math.min is to fix IE which reports 200% sometimes
fmodal.progress = Math.min(100, parseInt(100.0 * evt.loaded / evt.total));
// vm.loading = false;
// fmodal.loading = false;
});
}
}
}
function deleteFile(id) {
$ngConfirm({
title: 'حذف فایل',
content: '<span class="text-danger">{{"Are you sure about delete this item?"|translate}}</span>',
scope: $scope,
buttons: {
sayBoo: {
text: 'بله',
btnClass: 'btn-blue',
action: function (scope, button) {
vm.loading = true;
applicationService.deleteFile(vm.application.id, id)
.then(function () {
util.toast('success', 'فایل با موفقیت حذف شد.');
applicationService.getFiles(vm.application.id)
.then(function (data) {
vm.application.files = data;
vm.loading = false;
});
}, function () {
util.toast('error', 'خطا در حذف فایل');
vm.loading = false;
});
return true;
}
},
somethingElse: {
text: 'خیر',
btnClass: 'btn-orange',
action: function (scope, button) {
return true;
}
}
}
});
}
function deleteBanner() {
$ngConfirm({
title: 'حذف بنر',
content: '<span class="text-danger">{{"Are you sure about delete this item?"|translate}}</span>',
scope: $scope,
buttons: {
sayBoo: {
text: 'بله',
btnClass: 'btn-blue',
action: function (scope, button) {
vm.loading = true;
applicationService.deleteBanner(vm.application.id)
.then(function () {
util.toast('success', 'بنر با موفقیت حذف شد.');
applicationService.getFiles(vm.application.id)
.then(function () {
vm.application.banner = null;
vm.loading = false;
});
}, function () {
util.toast('error', 'خطا در حذف بنر');
vm.loading = false;
});
return true;
}
},
somethingElse: {
text: 'خیر',
btnClass: 'btn-orange',
action: function (scope, button) {
return true;
}
}
}
});
}
$scope.$watch(angular.bind(this, function () {
return this.picCropper;
}), function (newVal, oldVal) {
if(newVal) {
if(!oldVal || newVal.sourceImage !== oldVal.sourceImage){
console.log('Name changed to ', newVal , ' from ' , oldVal);
vm.sourceImage = new Image;
vm.sourceImage.onload = function () {
console.log('loaded');
};
vm.sourceImage.scr = newVal.sourceImage;
}
}
});
function initiateSuggestion() {
if(!vm.application.suggestionPriority){
vm.application.suggestionPriority = {appId : vm.application.id, priority: 0}
}
}
function getRatioDiff() {
return Math.abs((vm.bannerBounds.right - vm.bannerBounds.left) / (vm.bannerBounds.top - vm.bannerBounds.bottom) - (880 / 300));
}
function getActiveVersion() {
applicationService.getActiveVersion(vm.application.id).then(function (data) {
console.log('last version is', data.version);
vm.activeVersion = data.version;
vm.fileLoading = false;
}, function (failure) {
console.log(failure);
vm.fileLoading = false;
})
}
function setActiveVersion(file) {
vm.fileLoading = true;
if (file.version !== vm.activeVersion)
applicationService.setActiveVersion(vm.application.id, file.id).then(function () {
getActiveVersion();
})
}
function addGroup() {
if (!vm.group)
return;
applicationService.addGroup(vm.application.id, vm.group.id).then(function () {
if (!vm.application.groups)
vm.application.groups = [];
vm.application.groups.push(angular.copy(vm.group));
vm.group = null;
}, function (error) {
console.log(error);
if (error.data.exception === 'org.springframework.dao.DataIntegrityViolationException')
util.toast('error', "Group already exists for the application");
else
util.toast('error', "Error in adding group to application");
});
}
function removeGroup(group) {
if (!group)
return;
applicationService.removeGroup(vm.application.id, group.id).then(function () {
if (!vm.application.groups)
vm.application.groups = [];
var i = 0;
for (i; i < vm.application.groups.length; i++) {
if (vm.application.groups[i].id === group.id)
break;
}
vm.application.groups.splice(i, 1);
}, function (error) {
util.toast('error', "Error in removing group to application");
});
}
}
})();<file_sep># sbazaar
This is an Android App Store server which can support an Android app with the same goal with several features including varied searches, tagging, account management, rating and etc.
Springboot framework has been used as server side framework to implement logics and communincation with MySql database, and AngularJs is front-end framework to build a user friendly administration pannel.
<file_sep>package ir.saafta.web;
import ir.saafta.model.Advertisement;
import ir.saafta.model.Picture;
import ir.saafta.model.User;
import ir.saafta.repo.AdvertisementRepository;
import ir.saafta.repo.PictureRepository;
import ir.saafta.service.UserDetailManager;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
import java.text.ParseException;
import java.util.Date;
/**
* Created by reza on 11/14/16.
*/
@RestController
@RequestMapping("/ad")
public class AdvertisementController {
private final Logger logger = LoggerFactory.getLogger(this.getClass());
private final AdvertisementRepository advertisementRepository;
private final PictureRepository pictureRepository;
private final UserDetailManager userDetailManager;
@Autowired
public AdvertisementController(AdvertisementRepository advertisementRepository, PictureRepository pictureRepository,
UserDetailManager userDetailManager) {
this.advertisementRepository = advertisementRepository;
this.pictureRepository = pictureRepository;
this.userDetailManager = userDetailManager;
}
@RequestMapping(method = RequestMethod.GET)
public Page<Advertisement> get(Pageable pageable) {
return advertisementRepository.findAll(pageable);
}
@RequestMapping(value = "/current", method = RequestMethod.GET)
public Iterable<Advertisement> getCurrent() {
return advertisementRepository.findCurrentAdvertisement();
}
@RequestMapping(value = "/{id}", method = RequestMethod.GET)
public Advertisement get(@PathVariable Long id) {
return advertisementRepository.findOne(id);
}
@RequestMapping(method = RequestMethod.POST, consumes = "multipart/*")
public Advertisement create(MultipartFile file, String label,
@DateTimeFormat(pattern = "yyyy-MM-dd") Date fromDate,
@DateTimeFormat(pattern = "yyyy-MM-dd") Date toDate, String url, String username)
throws IOException, ParseException {
Advertisement advertisement = new Advertisement();
advertisement.setFromDate(fromDate);
advertisement.setToDate(toDate);
Picture picture = new Picture(file.getBytes(), label, "ad", file.getOriginalFilename());
pictureRepository.save(picture);
advertisement.setPicture(picture);
advertisement.setUrl(url);
advertisement.setSeenCount(0);
if (username != null) {
User user = (User) userDetailManager.loadUserByUsername(username);
advertisement.setUser(user);
}
return advertisementRepository.save(advertisement);
}
@RequestMapping(value = "/{id}", method = RequestMethod.PUT, consumes = "multipart/*")
public Advertisement setPicture(@PathVariable Long id, MultipartFile file, String label,
@DateTimeFormat(pattern = "yyyy-MM-dd") Date fromDate,
@DateTimeFormat(pattern = "yyyy-MM-dd") Date toDate, String url, String username) throws IOException, ParseException {
Advertisement advertisement = advertisementRepository.findOne(id);
advertisement.setFromDate(fromDate);
advertisement.setToDate(toDate);
if (advertisement.getPicture() != null) {
advertisement.getPicture().setLabel(label);
pictureRepository.save(advertisement.getPicture());
}
advertisement.setUrl(url);
if (username != null) {
User user = (User) userDetailManager.loadUserByUsername(username);
advertisement.setUser(user);
}
if (file != null && file.getBytes() != null) {
if (advertisement.getPicture() != null) {
Picture picture = advertisement.getPicture();
picture.setData(file.getBytes());
picture.setFormat(file.getOriginalFilename());
pictureRepository.save(picture);
} else {
Picture picture = new Picture(advertisement.getId(), file.getBytes(), advertisement.getTitle(), "ad", file.getOriginalFilename());
pictureRepository.save(picture);
}
}
return advertisementRepository.findOne(id);
}
@RequestMapping(value = "/seen/{id}", method = RequestMethod.PUT)
public Advertisement seenAd(@PathVariable Long id) {
Advertisement advertisement = advertisementRepository.findOne(id);
logger.info("Advertisement being seen with id " + id);
if (advertisement != null) {
Integer seenCount = advertisement.getSeenCount();
if (seenCount == null)
seenCount = 0;
seenCount++;
logger.info("seenCount is " + seenCount);
advertisement.setSeenCount(seenCount);
} else {
logger.info("Ad not found by id " + id);
}
return advertisementRepository.save(advertisement);
}
@RequestMapping(value = "/{id}", method = RequestMethod.DELETE)
public void delete(@PathVariable Long id) {
pictureRepository.delete(advertisementRepository.findOne(id).getPicture());
advertisementRepository.delete(id);
}
}
<file_sep>(function () {
'use strict';
angular
.module('app')
.config(config);
/** @ngInject */
function config($locationProvider, $logProvider, $httpProvider,
ADMdtpProvider, moment, toastrConfig, $uibTooltipProvider) {
// Enable log
$logProvider.debugEnabled(true);
// @desc add $http Interceptor
$httpProvider.interceptors.push('authInterceptor');
$httpProvider.defaults.withCredentials = true;
$httpProvider.defaults.headers['Content-Type'] = 'application/json;charset=UTF-8';
// disable # in url
// $locationProvider.html5Mode(true);
/////////////////////////////////
// Set options third-party lib //
/////////////////////////////////
ADMdtpProvider.setOptions({
autoClose: true,
calType: 'jalali',
dtpType: 'date',
format: 'YYYY-MM-DD',
freezeInput: true
});
// Set toastr options
angular.extend(toastrConfig, {
allowHtml: true,
closeButton: true,
closeHtml: '<button class="pull-left">×</button>',
extendedTimeOut: 500,
positionClass: 'toast-top-right',
preventDuplicates: false,
preventOpenDuplicates: true,
progressBar: true,
tapToDismiss: true,
// target: 'body',
timeOut: 2000
});
//moment
moment.loadPersian();
var parser = new UAParser();
var result = parser.getResult();
var touch = result.device && (result.device.type === 'tablet' || result.device.type === 'mobile');
console.log(result, touch);
if (touch) {
var options = {
trigger: 'dontTrigger' // default dummy trigger event to show tooltips
};
$uibTooltipProvider.options(options);
} else {
$uibTooltipProvider.options({
'appendToBody': true
})
}
}
})();
<file_sep>/*
SQLyog Ultimate v12.09 (64 bit)
MySQL - 5.7.10-log : Database - simorgh
*********************************************************************
*/
/*!40101 SET NAMES utf8 */;
/*!40101 SET SQL_MODE=''*/;
/*!40014 SET @OLD_UNIQUE_CHECKS=@@UNIQUE_CHECKS, UNIQUE_CHECKS=0 */;
/*!40014 SET @OLD_FOREIGN_KEY_CHECKS=@@FOREIGN_KEY_CHECKS, FOREIGN_KEY_CHECKS=0 */;
/*!40101 SET @OLD_SQL_MODE=@@SQL_MODE, SQL_MODE='NO_AUTO_VALUE_ON_ZERO' */;
/*!40111 SET @OLD_SQL_NOTES=@@SQL_NOTES, SQL_NOTES=0 */;
CREATE DATABASE /*!32312 IF NOT EXISTS*/`simorgh` /*!40100 DEFAULT CHARACTER SET utf8 */;
USE `simorgh`;
/*Table structure for table `access` */
DROP TABLE IF EXISTS `access`;
CREATE TABLE `access` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`code` varchar(50) DEFAULT NULL,
`description` text,
`label` varchar(50) CHARACTER SET utf8 COLLATE utf8_persian_ci DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=43 DEFAULT CHARSET=utf8;
/*Table structure for table `access_file` */
DROP TABLE IF EXISTS `access_file`;
CREATE TABLE `access_file` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`access_id` bigint(20) unsigned NOT NULL,
`file_id` bigint(20) NOT NULL,
PRIMARY KEY (`id`),
UNIQUE KEY `Unique_file_access` (`access_id`,`file_id`),
KEY `foreign_key_access_file_file` (`file_id`),
CONSTRAINT `access_file_access_to_access` FOREIGN KEY (`access_id`) REFERENCES `access` (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=52 DEFAULT CHARSET=utf8;
/*Table structure for table `advertisement` */
DROP TABLE IF EXISTS `advertisement`;
CREATE TABLE `advertisement` (
`id` bigint(20) NOT NULL,
`url` varchar(50) DEFAULT NULL,
`picture_id` bigint(20) DEFAULT NULL,
`user_id` varchar(50) CHARACTER SET utf8 COLLATE utf8_bin DEFAULT NULL,
`from_date` datetime DEFAULT NULL,
`to_date` datetime DEFAULT NULL,
`seen_count` binary(1) DEFAULT '0',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
/*Table structure for table `application` */
DROP TABLE IF EXISTS `application`;
CREATE TABLE `application` (
`id` bigint(20) unsigned NOT NULL,
`app_package` varchar(255) NOT NULL,
`fee` int(11) DEFAULT NULL,
`name` varchar(255) CHARACTER SET utf8 COLLATE utf8_persian_ci DEFAULT NULL,
`category_id` bigint(20) unsigned DEFAULT NULL,
`owner_id` bigint(20) unsigned DEFAULT NULL,
`logo_id` bigint(20) unsigned DEFAULT NULL,
`user_id` varchar(50) CHARACTER SET utf8 COLLATE utf8_bin DEFAULT NULL,
`create_date` datetime DEFAULT NULL,
`last_update` datetime DEFAULT NULL,
`specific_app` binary(1) DEFAULT '0',
`banner_id` bigint(20) unsigned DEFAULT NULL,
`new_app` binary(1) DEFAULT '0',
`last_file_id` bigint(20) unsigned DEFAULT NULL,
`recently_updated` binary(1) DEFAULT '0',
`installation_count` int(11) DEFAULT '0',
`in_app_payment` binary(1) DEFAULT '0',
`suggestion_priority` bigint(20) unsigned DEFAULT NULL,
`suggested_app` bigint(1) DEFAULT '0',
`disabled` binary(1) DEFAULT '0',
`incomplete` binary(1) DEFAULT '0',
`original_name` varchar(128) DEFAULT NULL,
`status` int(1) DEFAULT '1',
PRIMARY KEY (`id`),
KEY `foreign_key_application_picture` (`logo_id`),
KEY `foreign_key_application_category` (`category_id`),
KEY `foreign_key_application_picture_2` (`banner_id`),
KEY `foreign_key_application_file` (`last_file_id`),
KEY `foreign_key_application_owner` (`owner_id`),
KEY `unique` (`app_package`,`status`),
CONSTRAINT `foreign_key_application_category` FOREIGN KEY (`category_id`) REFERENCES `category` (`id`) ON UPDATE CASCADE,
CONSTRAINT `foreign_key_application_file_last_file` FOREIGN KEY (`last_file_id`) REFERENCES `file` (`id`) ON DELETE NO ACTION ON UPDATE CASCADE,
CONSTRAINT `foreign_key_application_owner` FOREIGN KEY (`owner_id`) REFERENCES `owner` (`id`) ON DELETE NO ACTION ON UPDATE CASCADE,
CONSTRAINT `foreign_key_application_picture` FOREIGN KEY (`logo_id`) REFERENCES `picture` (`id`) ON DELETE NO ACTION ON UPDATE CASCADE,
CONSTRAINT `foreign_key_application_picture_2` FOREIGN KEY (`banner_id`) REFERENCES `picture` (`id`) ON DELETE NO ACTION ON UPDATE CASCADE
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
/*Table structure for table `attempt` */
DROP TABLE IF EXISTS `attempt`;
CREATE TABLE `attempt` (
`session_id` varchar(100) NOT NULL,
`time` datetime DEFAULT NULL,
`username` varchar(50) DEFAULT NULL,
`captcha_passed` tinyint(1) DEFAULT '0',
`remote_address` varchar(30) DEFAULT NULL,
`device_info` varchar(255) DEFAULT NULL,
`captcha_value` varchar(30) DEFAULT NULL,
`true_password` tinyint(1) DEFAULT '0',
`count` int(8) unsigned DEFAULT '1',
PRIMARY KEY (`session_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
/*Table structure for table `authorities` */
DROP TABLE IF EXISTS `authorities`;
CREATE TABLE `authorities` (
`username` varchar(50) CHARACTER SET utf8 COLLATE utf8_bin NOT NULL,
`authority` varchar(45) NOT NULL,
PRIMARY KEY (`username`,`authority`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
/*Table structure for table `category` */
DROP TABLE IF EXISTS `category`;
CREATE TABLE `category` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`name` varchar(255) CHARACTER SET utf8 COLLATE utf8_persian_ci DEFAULT NULL,
`picture_id` bigint(20) unsigned DEFAULT NULL,
PRIMARY KEY (`id`),
UNIQUE KEY `category_name_unique` (`name`),
KEY `foreign_key_category_picture` (`picture_id`),
CONSTRAINT `foreign_key_category_picture` FOREIGN KEY (`picture_id`) REFERENCES `picture` (`id`) ON DELETE CASCADE ON UPDATE CASCADE
) ENGINE=InnoDB AUTO_INCREMENT=21 DEFAULT CHARSET=utf8;
/*Table structure for table `comment` */
DROP TABLE IF EXISTS `comment`;
CREATE TABLE `comment` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`content` varchar(255) DEFAULT NULL,
`content_id` bigint(20) unsigned DEFAULT NULL,
`date` datetime DEFAULT NULL,
`user_id` varchar(45) CHARACTER SET utf8 COLLATE utf8_bin DEFAULT NULL,
`approved` binary(1) DEFAULT '0',
PRIMARY KEY (`id`),
KEY `foreign_key_comment_contetn` (`content_id`),
CONSTRAINT `foreign_key_comment_contetn` FOREIGN KEY (`content_id`) REFERENCES `content` (`id`) ON DELETE CASCADE ON UPDATE CASCADE
) ENGINE=InnoDB AUTO_INCREMENT=3 DEFAULT CHARSET=utf8;
/*Table structure for table `content` */
DROP TABLE IF EXISTS `content`;
CREATE TABLE `content` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`description` text,
`seen_count` int(11) unsigned NOT NULL DEFAULT '0',
`title` varchar(255) CHARACTER SET utf8 COLLATE utf8_persian_ci DEFAULT NULL,
`type` varchar(255) DEFAULT NULL,
`DTYPE` varchar(45) DEFAULT NULL,
`rating` double DEFAULT '0',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=100 DEFAULT CHARSET=utf8;
/*Table structure for table `content_group` */
DROP TABLE IF EXISTS `content_group`;
CREATE TABLE `content_group` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`group_id` bigint(20) unsigned NOT NULL,
`content_id` bigint(20) unsigned NOT NULL,
PRIMARY KEY (`id`),
UNIQUE KEY `content_group_unique` (`group_id`,`content_id`),
KEY `content_id_fk` (`content_id`),
CONSTRAINT `content_id_fk` FOREIGN KEY (`content_id`) REFERENCES `content` (`id`) ON DELETE CASCADE ON UPDATE CASCADE,
CONSTRAINT `group_id_fk` FOREIGN KEY (`group_id`) REFERENCES `user_group` (`id`) ON DELETE CASCADE ON UPDATE CASCADE
) ENGINE=InnoDB AUTO_INCREMENT=38 DEFAULT CHARSET=utf8;
/*Table structure for table `deleted_user` */
DROP TABLE IF EXISTS `deleted_user`;
CREATE TABLE `deleted_user` (
`username` varchar(50) CHARACTER SET utf8 COLLATE utf8_bin NOT NULL,
`deletion_date` datetime NOT NULL,
`deleted_by` varchar(50) CHARACTER SET utf8 COLLATE utf8_bin NOT NULL,
`credit` int(11) DEFAULT NULL,
`score` int(11) DEFAULT NULL,
PRIMARY KEY (`username`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
/*Table structure for table `event` */
DROP TABLE IF EXISTS `event`;
CREATE TABLE `event` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`time` datetime NOT NULL,
`action` varchar(50) NOT NULL,
`username` varchar(50) CHARACTER SET utf8 COLLATE utf8_bin DEFAULT NULL,
`remote_addr` varchar(30) DEFAULT NULL,
`host` varchar(50) DEFAULT NULL,
`session_id` varchar(100) DEFAULT NULL,
`cookie` varchar(500) DEFAULT NULL,
`method` varchar(10) DEFAULT NULL,
`params` text,
`body` text,
`user_agent` text,
`path` varchar(50) DEFAULT NULL,
`status` varchar(3) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=10046 DEFAULT CHARSET=utf8;
/*Table structure for table `feedback` */
DROP TABLE IF EXISTS `feedback`;
CREATE TABLE `feedback` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`date` datetime DEFAULT NULL,
`liked` tinyint(1) DEFAULT '0',
`disliked` tinyint(1) DEFAULT '0',
`rate` double DEFAULT '0',
`content_id` bigint(20) unsigned DEFAULT NULL,
`username` varchar(50) CHARACTER SET utf8 COLLATE utf8_bin DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `foreign_key_feedback_content` (`content_id`),
CONSTRAINT `foreign_key_feedback_content` FOREIGN KEY (`content_id`) REFERENCES `content` (`id`) ON DELETE CASCADE ON UPDATE CASCADE
) ENGINE=InnoDB AUTO_INCREMENT=8 DEFAULT CHARSET=utf8;
/*Table structure for table `file` */
DROP TABLE IF EXISTS `file`;
CREATE TABLE `file` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`application_id` bigint(20) DEFAULT NULL,
`data` longblob,
`description` text,
`name` varchar(255) DEFAULT NULL,
`registration_date` datetime DEFAULT NULL,
`size` int(11) DEFAULT NULL,
`version` varchar(255) DEFAULT NULL,
`minimum_android_version` varchar(255) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=28 DEFAULT CHARSET=utf8;
/*Table structure for table `news` */
DROP TABLE IF EXISTS `news`;
CREATE TABLE `news` (
`id` bigint(20) unsigned NOT NULL,
`body` text,
`main_picture_id` bigint(20) DEFAULT NULL,
`date` datetime NOT NULL,
`writer` varchar(60) DEFAULT NULL,
`lead` varchar(200) DEFAULT NULL,
PRIMARY KEY (`id`),
UNIQUE KEY `id_UNIQUE` (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
/*Table structure for table `owner` */
DROP TABLE IF EXISTS `owner`;
CREATE TABLE `owner` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`name` varchar(255) CHARACTER SET utf8 COLLATE utf8_persian_ci DEFAULT NULL,
`url` varchar(255) DEFAULT NULL,
`email` varchar(50) DEFAULT NULL,
`phone_number` varchar(15) DEFAULT NULL,
`type` int(1) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=15 DEFAULT CHARSET=utf8;
/*Table structure for table `picture` */
DROP TABLE IF EXISTS `picture`;
CREATE TABLE `picture` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`content_id` bigint(20) unsigned DEFAULT NULL,
`data` longblob,
`label` varchar(255) DEFAULT NULL,
`type` varchar(255) DEFAULT NULL,
`published` tinyint(1) DEFAULT NULL,
`format` varchar(64) DEFAULT NULL,
`application_status` int(1) DEFAULT '1',
PRIMARY KEY (`id`),
KEY `foreign_key_picture_content` (`content_id`),
CONSTRAINT `foreign_key_picture_content` FOREIGN KEY (`content_id`) REFERENCES `content` (`id`) ON DELETE CASCADE ON UPDATE CASCADE
) ENGINE=InnoDB AUTO_INCREMENT=388 DEFAULT CHARSET=utf8;
/*Table structure for table `role` */
DROP TABLE IF EXISTS `role`;
CREATE TABLE `role` (
`authority` varchar(50) NOT NULL,
`caption` varchar(50) CHARACTER SET utf8 COLLATE utf8_persian_ci DEFAULT NULL,
PRIMARY KEY (`authority`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
/*Table structure for table `session` */
DROP TABLE IF EXISTS `session`;
CREATE TABLE `session` (
`session_id` varchar(100) NOT NULL,
`username` varchar(50) CHARACTER SET utf8 COLLATE utf8_bin NOT NULL,
`creation_time` datetime DEFAULT NULL,
`finish_time` datetime DEFAULT NULL,
`status` int(1) DEFAULT NULL,
`last_request` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP,
`remote_addr` varchar(30) DEFAULT NULL,
`device_info` varchar(255) DEFAULT NULL,
PRIMARY KEY (`session_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
/*Table structure for table `spring_session` */
DROP TABLE IF EXISTS `spring_session`;
CREATE TABLE `spring_session` (
`PRIMARY_ID` char(36) DEFAULT NULL,
`SESSION_ID` char(36) NOT NULL,
`CREATION_TIME` bigint(20) NOT NULL,
`LAST_ACCESS_TIME` bigint(20) NOT NULL,
`MAX_INACTIVE_INTERVAL` int(11) NOT NULL,
`EXPIRY_TIME` bigint(20) DEFAULT NULL,
`PRINCIPAL_NAME` varchar(100) DEFAULT NULL,
PRIMARY KEY (`SESSION_ID`),
UNIQUE KEY `SPRING_SESSION_IX1` (`SESSION_ID`),
KEY `SPRING_SESSION_IX2` (`EXPIRY_TIME`),
KEY `SPRING_SESSION_IX3` (`PRINCIPAL_NAME`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8 ROW_FORMAT=DYNAMIC;
/*Table structure for table `spring_session_attributes` */
DROP TABLE IF EXISTS `spring_session_attributes`;
CREATE TABLE `spring_session_attributes` (
`SESSION_ID` char(36) NOT NULL,
`ATTRIBUTE_NAME` varchar(200) NOT NULL,
`ATTRIBUTE_BYTES` blob NOT NULL,
PRIMARY KEY (`ATTRIBUTE_NAME`,`SESSION_ID`),
KEY `SPRING_SESSION_ATTRIBUTES_FK` (`SESSION_ID`),
CONSTRAINT `SPRING_SESSION_ATTRIBUTES_FK` FOREIGN KEY (`SESSION_ID`) REFERENCES `spring_session` (`SESSION_ID`) ON DELETE CASCADE
) ENGINE=InnoDB DEFAULT CHARSET=utf8 ROW_FORMAT=DYNAMIC;
/*Table structure for table `suggestion_priority` */
DROP TABLE IF EXISTS `suggestion_priority`;
CREATE TABLE `suggestion_priority` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`app_id` bigint(20) unsigned DEFAULT NULL,
`priority` int(2) unsigned DEFAULT '0',
PRIMARY KEY (`id`),
KEY `suggestion_priority_application` (`app_id`),
CONSTRAINT `suggestion_priority_application` FOREIGN KEY (`app_id`) REFERENCES `application` (`id`) ON DELETE CASCADE ON UPDATE CASCADE
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
/*Table structure for table `transaction` */
DROP TABLE IF EXISTS `transaction`;
CREATE TABLE `transaction` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`date` datetime DEFAULT NULL,
`amount` int(11) DEFAULT NULL,
`type` varchar(20) DEFAULT NULL,
`app_id` bigint(20) unsigned DEFAULT NULL,
`transaction_id` bigint(20) unsigned DEFAULT NULL,
`reference_id` bigint(20) unsigned DEFAULT NULL,
`username` varchar(50) CHARACTER SET utf8 COLLATE utf8_bin DEFAULT NULL,
`comment` varchar(1024) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `transaction_fk2_appId` (`app_id`),
CONSTRAINT `transaction_fk2_appId` FOREIGN KEY (`app_id`) REFERENCES `content` (`id`) ON DELETE NO ACTION ON UPDATE CASCADE
) ENGINE=InnoDB AUTO_INCREMENT=18 DEFAULT CHARSET=utf8;
/*Table structure for table `user` */
DROP TABLE IF EXISTS `user`;
CREATE TABLE `user` (
`username` varchar(50) CHARACTER SET utf8 COLLATE utf8_bin NOT NULL,
`password` varchar(60) NOT NULL,
`enabled` tinyint(4) DEFAULT '1',
`name` varchar(45) CHARACTER SET utf8 COLLATE utf8_persian_ci DEFAULT NULL,
`family` varchar(45) CHARACTER SET utf8 COLLATE utf8_persian_ci DEFAULT NULL,
`address` text,
`mobile_phone` varchar(13) DEFAULT NULL,
`mobile_phone_two` varchar(13) DEFAULT NULL,
`mobile_phone_three` varchar(13) DEFAULT NULL,
`phone` varchar(14) DEFAULT NULL,
`postal_code` varchar(45) DEFAULT NULL,
`email` varchar(45) DEFAULT NULL,
`profile_id` bigint(20) unsigned DEFAULT NULL,
`credit` int(11) DEFAULT '0',
`score` int(11) DEFAULT '0',
`imei` varchar(40) DEFAULT NULL,
`user_group_id` bigint(20) unsigned DEFAULT NULL,
PRIMARY KEY (`username`),
KEY `user_fk1_profile_pic` (`profile_id`),
KEY `user_fk2_group` (`user_group_id`),
CONSTRAINT `user_fk1_profile_pic` FOREIGN KEY (`profile_id`) REFERENCES `picture` (`id`) ON DELETE NO ACTION ON UPDATE CASCADE,
CONSTRAINT `user_fk2_group` FOREIGN KEY (`user_group_id`) REFERENCES `user_group` (`id`) ON DELETE NO ACTION ON UPDATE CASCADE
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
/*Table structure for table `user_app` */
DROP TABLE IF EXISTS `user_app`;
CREATE TABLE `user_app` (
`id` bigint(20) unsigned NOT NULL,
`install_date` datetime DEFAULT NULL,
`uninstall_date` datetime DEFAULT NULL,
`current_version` varchar(255) DEFAULT NULL,
`bookmarked` binary(1) DEFAULT '0',
KEY `user_app_user_content_id_fk` (`id`),
CONSTRAINT `user_app_user_content_id_fk` FOREIGN KEY (`id`) REFERENCES `user_content` (`id`) ON DELETE CASCADE ON UPDATE CASCADE
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
/*Table structure for table `user_content` */
DROP TABLE IF EXISTS `user_content`;
CREATE TABLE `user_content` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`username` varchar(50) CHARACTER SET utf8 COLLATE utf8_bin DEFAULT NULL,
`content_id` bigint(20) unsigned DEFAULT NULL,
`phone_number` varchar(14) DEFAULT NULL,
`rating` double DEFAULT '-1',
`install_date` datetime DEFAULT NULL,
`uninstall_date` datetime DEFAULT NULL,
`current_version` varchar(255) DEFAULT NULL,
`bookmarked` binary(1) DEFAULT NULL,
`bought` binary(1) DEFAULT '0',
`session` varchar(100) DEFAULT NULL,
PRIMARY KEY (`id`),
UNIQUE KEY `user_content_session_unique` (`session`,`content_id`),
KEY `user_content_content_id_fk` (`content_id`),
CONSTRAINT `user_content_content_id_fk` FOREIGN KEY (`content_id`) REFERENCES `content` (`id`) ON DELETE CASCADE ON UPDATE CASCADE
) ENGINE=InnoDB AUTO_INCREMENT=137 DEFAULT CHARSET=utf8;
/*Table structure for table `user_group` */
DROP TABLE IF EXISTS `user_group`;
CREATE TABLE `user_group` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`title` varchar(50) CHARACTER SET utf8 COLLATE utf8_persian_ci NOT NULL,
`logo_id` bigint(20) unsigned DEFAULT NULL,
`default_group` binary(1) DEFAULT NULL,
PRIMARY KEY (`id`),
UNIQUE KEY `user_group_name_unique` (`title`)
) ENGINE=InnoDB AUTO_INCREMENT=4 DEFAULT CHARSET=utf8;
/*Table structure for table `user_user_group` */
DROP TABLE IF EXISTS `user_user_group`;
CREATE TABLE `user_user_group` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`group_id` bigint(20) unsigned NOT NULL,
`username` varchar(50) CHARACTER SET utf8 COLLATE utf8_bin NOT NULL,
PRIMARY KEY (`id`),
UNIQUE KEY `unique_relation` (`group_id`,`username`),
KEY `user_group_username_fk` (`username`),
CONSTRAINT `user_group_groupId_fk` FOREIGN KEY (`group_id`) REFERENCES `user_group` (`id`) ON DELETE CASCADE ON UPDATE CASCADE,
CONSTRAINT `user_group_username_fk` FOREIGN KEY (`username`) REFERENCES `user` (`username`) ON DELETE CASCADE ON UPDATE CASCADE
) ENGINE=InnoDB AUTO_INCREMENT=29 DEFAULT CHARSET=utf8;
/*!40101 SET SQL_MODE=@OLD_SQL_MODE */;
/*!40014 SET FOREIGN_KEY_CHECKS=@OLD_FOREIGN_KEY_CHECKS */;
/*!40014 SET UNIQUE_CHECKS=@OLD_UNIQUE_CHECKS */;
/*!40111 SET SQL_NOTES=@OLD_SQL_NOTES */;
<file_sep>package ir.saafta.service;
import ir.saafta.model.Authority;
import ir.saafta.model.User;
import ir.saafta.repo.UserRepository;
import org.apache.catalina.servlet4preview.http.HttpServletRequest;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.core.userdetails.UsernameNotFoundException;
import org.springframework.security.provisioning.UserDetailsManager;
import org.springframework.stereotype.Component;
/**
* Created by reza on 11/12/16.
*/
@Component
public class UserDetailManager implements UserDetailsManager {
private final UserRepository userRepository;
@Autowired
private HttpServletRequest request;
@Autowired
private LoginAttemptService loginAttemptService;
@Autowired
public UserDetailManager(UserRepository userRepository) {
this.userRepository = userRepository;
}
@Override
public void createUser(UserDetails user) {
User theUser = (User) user;
if (this.loadUserByUsername(user.getUsername()) == null)
userRepository.save(theUser);
}
@Override
public void updateUser(UserDetails user) {
User theUser = (User) user;
userRepository.save(theUser);
}
public User save(User user) {
return userRepository.save(user);
}
@Override
public void deleteUser(String username) {
userRepository.delete(username);
}
@Override
public void changePassword(String oldPassword, String newPassword) {
}
@Override
public boolean userExists(String username) {
return userRepository.findOne(username) != null;
}
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
String ip = getClientIP();
String dId = getDeviceId();
if (loginAttemptService.isBlocked(ip + dId)) {
throw new RuntimeException("blocked");
}
return userRepository.findOne(username);
}
public Long count() {
return userRepository.count();
}
public UserDetails enableUser(String username) {
User user = (User) loadUserByUsername(username);
user.setEnabled(true);
return userRepository.save(user);
}
public UserDetails disableUser(String username) {
User user = (User) loadUserByUsername(username);
user.setEnabled(false);
return userRepository.save(user);
}
public boolean hasAuthority(UserDetails user, String authority) {
for (GrantedAuthority auth : user.getAuthorities()) {
if (auth.getAuthority().equals(authority))
return true;
}
return false;
}
public boolean hasAuthority(Authentication authentication, String authority) {
User user = (User) this.loadUserByUsername(authentication.getName());
for (GrantedAuthority auth : user.getAuthorities()) {
if (auth.getAuthority().equals(authority))
return true;
}
return false;
}
public boolean hasAuthorities(UserDetails user, Iterable<String> authorities) {
for (String auth : authorities) {
if (hasAuthority(user, auth))
return true;
}
return false;
}
public boolean hasAuthorities(Authentication authentication, Iterable<String> authorities) {
User user = (User) this.loadUserByUsername(authentication.getName());
for (String auth : authorities) {
if (hasAuthority(user, auth))
return true;
}
return false;
}
private String getClientIP() {
String xfHeader = request.getHeader("X-Forwarded-For");
if (xfHeader == null) {
return request.getRemoteAddr();
}
return xfHeader.split(",")[0];
}
private String getDeviceId() {
return request.getHeader("User-Agent");
}
}
<file_sep>package ir.saafta.conf;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Profile;
import springfox.documentation.builders.PathSelectors;
import springfox.documentation.builders.RequestHandlerSelectors;
import springfox.documentation.service.ApiInfo;
import springfox.documentation.spi.DocumentationType;
import springfox.documentation.spring.web.plugins.Docket;
import springfox.documentation.swagger2.annotations.EnableSwagger2;
/**
* User: <NAME>
*/
@Profile("!production")
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.any())
.paths(PathSelectors.regex("/.*"))
.build()
.apiInfo(new ApiInfo(
"Simorgh Market Application",
"Simorgh Market is an Android app-store which focuses on security apps",
"version 1.0.0",
"API TOS",
"<EMAIL>",
"API License",
"API License URL"
));
}
}
<file_sep>/**
* Created by reza on 11/14/16.
*/
(function () {
'use strict';
angular
.module('app')
.config(routeConfig);
/** @ngInject */
function routeConfig($stateProvider) {
$stateProvider
.state('root.userManagement', {
controller: 'UserController',
controllerAs: 'vm',
data: {
roles: [
"admin"
]
},
resolve: {},
templateUrl: 'app/components/user/user.html',
url: '/user-management'
})
;
}
})();
<file_sep>(function () {
'use strict';
angular
.module('app')
.factory('homeService', homeService);
/** @ngInject */
function homeService($log, $http, host) {
var base = host + 'dashboard/';
var service = {
getDashboard: getDashboard,
getMyDashboard: getMyDashboard
};
return service;
////////////////
function getDashboard(params) {
return $http.get(base, {params: params})
.then(successCallback);
}
function getMyDashboard(params) {
var url = base + 'me';
return $http.get(url, {params: params})
.then(successCallback);
}
function successCallback(response) {
return response.data;
}
}
})();<file_sep>/**
* Created by Reza on 11/30/2016.
*/
(function () {
'use strict';
angular
.module('app')
.factory('fileService', fileService);
/** @ngInject */
function fileService($log, $http, host) {
var base = host + 'file/';
var service = {
updateFile: updateFile,
deleteFile: deleteFile
};
return service;
function successCallback(response) {
return response.data;
}
////////////////
function updateFile(file) {
return $http.put(base, file)
.then(successCallback);
}
function deleteFile(id) {
var url = base + id;
return $http.delete(url)
.then(successCallback);
}
}
})();<file_sep>(function () {
'use strict';
angular
.module('auth')
.controller('ForgetPassController', ForgetPassController);
/** @ngInject */
function ForgetPassController($log) {
var vm = this;
vm.title = 'ForgetPassController';
activate();
////////////////
function activate() {
$log.debug('forget pass module ativated.');
}
}
})();
<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('CreateUpdateUserController', CreateUpdateUserController);
/** @ngInject */
function CreateUpdateUserController($log, toastr, userService, $stateParams, $scope, $timeout, $state) {
var vm = this;
vm.title = 'CreateUpdateUserController';
vm.method = $stateParams.method;
vm.user = {
username: $stateParams.username,
authorities: []
};
vm.save = save;
vm.reset = reset;
vm.resetPassword = <PASSWORD>;
activate();
////////////////
function activate() {
$log.debug('User module activated');
getAuthorities().then(getUser);
}
function save() {
vm.user.authorities = [];
vm.userAuthorities.forEach(function (authority) {
vm.user.authorities.push({
authority: authority.authority,
username: vm.user.username
})
});
switch (vm.method) {
case 'create':
userService.createUser(vm.user).then(function () {
toastr.success('کاربر با موفقیت ایجاد شد.');
$timeout(function () {
$state.go('root.user');
}, 3000);
}, function () {
toastr.error('خطا در ایجاد کاربر!');
});
break;
case 'update':
userService.updateUser(vm.user).then(function () {
toastr.success('کابر با موفقیت به روزرسانی شد.')
}, function () {
toastr.error('خطا در به روزرسانی کاربر!');
});
}
}
function getAuthorities() {
return userService.getAuthorities().then(function (data) {
vm.authorities = data;
})
}
function getCaption(authority) {
for (var i = 0; i < vm.authorities.length; i++) {
if (vm.authorities[i].authority == authority)
return vm.authorities[i].caption;
}
}
function reset() {
switch (vm.method) {
case 'create':
vm.user = {
authorities: []
};
console.log('method');
break;
case 'update':
getUser();
}
}
function getUser() {
vm.userAuthorities = [];
if (vm.user.username) {
userService.getUser(vm.user.username).then(function (data) {
vm.user = data;
// console.log(data);
vm.passwordCheck = data.<PASSWORD>;
vm.user.authorities.forEach(function (authority) {
vm.userAuthorities.push({
"authority": authority.authority,
"caption": getCaption(authority.authority)
})
})
});
}
}
function resetPassword() {
userService.resetPassword(vm.user.username, vm.user.password).then(function () {
toastr.success('کلمه عبور با موفقیت به روزرسانی شد.')
}, function () {
toastr.error('خطا در به روزرسانی کلمه عبور!');
});
}
var stillClearPassword = true;
$scope.$watch(angular.bind(this, function () {
return this.user.password;
}), function (newVal) {
if (stillClearPassword && newVal !== undefined && newVal !== null) {
vm.user.password = '';
stillClearPassword = false;
}
});
}
})();<file_sep>angular.module('bootstrapWysiwyg', []).directive('bootstrapWysiwyg', function () {
'use strict';
return {
restrict: 'AE',
scope: {
'toolbar': '=',
'useLineBreaks': '=',
'parserRules': '=',
'locale': '=',
'shortcuts': '='
},
link: function (scope, element) {
if (typeof scope.toolbar === 'string') {
scope.toolbar = JSON.parse(scope.toolbar);
}
if (typeof scope.parserRules === 'string') {
scope.toolbar = JSON.parse(scope.toolbar);
}
if (typeof scope.shortcuts === 'string') {
scope.toolbar = JSON.parse(scope.toolbar);
}
$.fn.wysihtml5.defaultOptions = {
'toolbar': {
'fa': true
}
};
$(element).wysihtml5(scope);
}
};
});
<file_sep>(function () {
'use strict';
angular
.module('app')
.factory('util', util);
/** @ngInject */
function util($log, $translate, toastr) {
var colors = [
"red",
"blue",
"cyan",
"light-blue",
"lime",
"pink",
"green",
"purple",
"yellow",
"deep-purple",
"indigo",
"teal",
"light-green",
"blue-grey",
"amber",
"orange",
"deep-orange",
"brown",
"grey",
"shades"];
var service = {
compareObjects: compareObjects,
toast: toast,
transformRequest: transformRequest,
colors: colors
};
return service;
////////////////
function compareObjects(object1, object2) {
return angular.toJson(object1) === angular.toJson(object2);
}
function toast(type, text, title) {
$translate(text).then(toaster, toaster);
console.log('toast', type, text, title);
function toaster(msg) {
console.log('toaster', msg);
switch (type) {
case 'error':
toastr.error(msg);
$log.error(title);
break;
case 'info':
toastr.info(msg);
break;
case 'success':
toastr.success(msg);
break;
case 'warn':
toastr.warning(msg);
$log.warn(title);
break;
default:
toastr.info(msg);
}
}
}
function transformRequest(data) {
var formData = new FormData();
angular.forEach(data, function (value, key) {
formData.append(key, value);
});
return formData;
}
}
})();
<file_sep>(function () {
'use strict';
angular
.module('app')
.factory('categoryService', categoryService);
/** @ngInject */
function categoryService($log, $http, host, Upload) {
var base = host + 'category/';
var service = {
getCategories: getCategories,
getAllCategories: getAllCategories,
getCategory: getCategory,
createCategory: createCategory,
updateCategory: updateCategory,
deleteCategory: deleteCategory
};
return service;
function successCallback(response) {
return response.data;
}
////////////////
function createCategory(name, dataUrl) {
return Upload.upload({
url: base,
data: {
pic: Upload.dataUrltoBlob(dataUrl, 'image.png'),
name: name
},
method: 'POST'
});
// return $http.post(base, category)
// .then(successCallback);
}
function getCategories(params) {
return $http.get(base, {params: params})
.then(successCallback);
}
function getAllCategories() {
var url = base + 'all';
return $http.get(url)
.then(successCallback);
}
function getCategory(id) {
var url = base + id;
return $http.get(url)
.then(successCallback);
}
function updateCategory(id, name, dataUrl) {
console.log('updateCategory ', id);
return Upload.upload({
url: base + id,
data: {
pic: dataUrl ? Upload.dataUrltoBlob(dataUrl, 'image.png') : null,
name: name
},
method: 'PUT'
}).then(successCallback);
}
function deleteCategory(id) {
var url = base + id;
return $http.delete(url)
.then(successCallback);
}
}
})();<file_sep>(function () {
'use strict';
angular
.module('app')
.factory('bannerService', bannerService);
/** @ngInject */
function bannerService($log, $http, host, Upload) {
var base = host + 'banner/';
var service = {
getBanners: getBanners,
getAllBanners: getAllBanners,
getBanner: getBanner,
createBanner: createBanner,
updateBanner: updateBanner,
deleteBanner: deleteBanner,
publishBanner: publishBanner
};
return service;
function successCallback(response) {
return response.data;
}
////////////////
function createBanner(label, dataUrl) {
return Upload.upload({
url: base,
data: {
file: Upload.dataUrltoBlob(dataUrl, 'image.jpg'),
label: label
},
method: 'POST'
});
// return $http.post(base, banner)
// .then(successCallback);
}
function getBanners(params) {
return $http.get(base, {params: params})
.then(successCallback);
}
function getAllBanners() {
var url = base + 'all';
return $http.get(url)
.then(successCallback);
}
function getBanner(id) {
var url = base + id;
return $http.get(url)
.then(successCallback);
}
function updateBanner(id, caption) {
console.log('updateBanner ', id);
var url = base + id;
return $http.put(url, {}, {params: {caption: caption}}).then(successCallback);
}
function deleteBanner(id) {
var url = base + id;
return $http.delete(url)
.then(successCallback);
}
function publishBanner(id, publish) {
console.log('publishBanner', id, publish);
var url = base + id + '/publish';
return $http.put(url, {},
{
params: {
'publish': publish
}
});
}
}
})();<file_sep>package ir.saafta.model;
import javax.persistence.Entity;
import javax.persistence.Id;
import java.util.Date;
@Entity
public class Attempt {
@Id
private String sessionId;
private Date time;
private String username;
private boolean captchaPassed;
private String remoteAddress;
private String deviceInfo;
private String captchaValue;
private boolean truePassword;
private Integer count;
public Attempt() {
this.count = 1;
}
public Attempt(String captchaValue, String sessionId, String remoteAddress, String deviceInfo) {
this.sessionId = sessionId;
this.remoteAddress = remoteAddress;
this.deviceInfo = deviceInfo;
this.captchaValue = captchaValue;
this.count = 1;
}
public String getSessionId() {
return sessionId;
}
public void setSessionId(String sessionId) {
this.sessionId = sessionId;
}
public Date getTime() {
return time;
}
public void setTime(Date time) {
this.time = time;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public boolean isCaptchaPassed() {
return captchaPassed;
}
public void setCaptchaPassed(boolean captchaPassed) {
this.captchaPassed = captchaPassed;
}
public String getRemoteAddress() {
return remoteAddress;
}
public void setRemoteAddress(String remoteAddress) {
this.remoteAddress = remoteAddress;
}
public String getDeviceInfo() {
return deviceInfo;
}
public void setDeviceInfo(String deviceInfo) {
this.deviceInfo = deviceInfo;
}
public String getCaptchaValue() {
return captchaValue;
}
public void setCaptchaValue(String captchaValue) {
this.captchaValue = captchaValue;
}
public boolean isTruePassword() {
return truePassword;
}
public void setTruePassword(boolean truePassword) {
this.truePassword = truePassword;
}
public Integer getCount() {
return count;
}
public void setCount(Integer count) {
this.count = count;
}
public void increaseCount() {
if (this.count == null)
this.count = 1;
this.count++;
}
}
<file_sep>package ir.saafta.repo;
import ir.saafta.model.Content;
import ir.saafta.model.User;
import ir.saafta.model.UserContent;
import org.springframework.data.jpa.repository.Modifying;
import org.springframework.data.jpa.repository.Query;
import org.springframework.data.repository.PagingAndSortingRepository;
import org.springframework.data.repository.query.Param;
/**
* Created by Reza on 1/20/2017.
*/
public interface UserContentRepository extends PagingAndSortingRepository<UserContent, Long> {
UserContent findByUserAndContent(User user, Content content);
Iterable<UserContent> findByContent(Content content);
@Modifying(clearAutomatically = true)
@Query("update UserContent userContent set userContent.currentVersion =:currentVersion where userContent.currentVersion =:oldVersion")
void updateVersions(@Param("currentVersion") String currentVersion, @Param("oldVersion") String oldVersion);
}
<file_sep>(function () {
'use strict';
angular
.module('app')
.factory('newsService', newsService);
/** @ngInject */
function newsService($log, $http, host, Upload) {
var base = host + 'news/';
var service = {
getNewsList: getNewsList,
getAllNewsList: getAllNewsList,
getNews: getNews,
createNews: createNews,
mainPicture: mainPicture,
updateNews: updateNews,
deleteNews: deleteNews
};
return service;
function successCallback(response) {
return response.data;
}
////////////////
function createNews(news) {
return $http.post(base, news);
}
function mainPicture(id, dataUrl) {
var url = base + id + '/mainPicture';
return Upload.upload({
url: url,
data: {
pic: Upload.dataUrltoBlob(dataUrl, 'image.png')
},
method: 'POST'
}).then(successCallback);
}
function getNewsList(params) {
return $http.get(base, {params: params})
.then(successCallback);
}
function getAllNewsList() {
var url = base + 'all';
return $http.get(url)
.then(successCallback);
}
function getNews(id) {
var url = base + id;
var params = {
manage: true
};
return $http.get(url, {params: params})
.then(successCallback);
}
function updateNews(news) {
var url = base + news.id;
return $http.put(url, news).then(successCallback);
}
function deleteNews(id) {
var url = base + id;
return $http.delete(url)
.then(successCallback);
}
}
})();<file_sep>package ir.saafta.repo;
import ir.saafta.dto.ApplicationStatus;
import ir.saafta.model.Picture;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.data.repository.PagingAndSortingRepository;
import java.util.ArrayList;
import java.util.Collection;
/**
* Created by reza on 11/13/16.
*/
public interface PictureRepository extends PagingAndSortingRepository<Picture, Long> {
Iterable<Picture> findByPublished(Boolean published);
// ArrayList<Picture> findByPublishedAndLuncherAndNonLuncher(Boolean published, Boolean luncher, Boolean nonLuncher);
//
// ArrayList<Picture> findByPublishedAndLuncher(Boolean published, Boolean luncher);
//
// ArrayList<Picture> findByPublishedAndNonLuncher(Boolean published, Boolean nonLuncher);
ArrayList<Picture> findByPublishedAndApplicationStatusIn(Boolean published, Collection<ApplicationStatus> statuses);
Page<Picture> findByTypeEquals(String type, Pageable pageable);
}
<file_sep>(function () {
'use strict';
angular
.module('auth')
.factory('authInterceptor', authInterceptor);
/** @ngInject */
function authInterceptor($log, $q, $window, $state, host) {
var Authentication = {
// request: onRequest,
response: onResponse,
responseError: onResponseError
};
return Authentication;
////////////////
function onRequest(config) {
var deferred = $q.defer();
// promise that should abort the request when resolved.
config.timeout = deferred.promise;
config.cancel = deferred;
return config;
}
function onResponse(response) {
var x = typeof response.data === 'string' &&
response.data.indexOf('session has been expired') > -1;
if (x) {
location.reload();
return response || $q.when(response);
}
return response || $q.when(response);
}
function onResponseError(response) {
if (response.status === 401) {
// user is not authenticated
// console.error('user is not authenticated', response);
// $window.location.href = '/#!/login';
$state.go('login');
}
if (response.status === 403) {
// user is not authenticated
// $log.warn('operation forbidden', response);
}
if (response.status === 500) {
// console.log('500', response);
if (response.data.message === 'Invalid token.') {
// $state.go('login');
window.location.reload();
}
}
// if (response.status === 404) {
// $log.error('request is not found', response);
// $window.location.href = '/#/404';
// }
return $q.reject(response);
}
}
})();
<file_sep>(function () {
'use strict';
angular
.module('app')
.factory('userService', userService);
/** @ngInject */
function userService($log, $http, host) {
var base = host + 'user/';
var service = {
getUsers: getUsers
};
return service;
////////////////
function getUsers(params) {
return $http.get(base, {params: params})
.then(successCallback);
}
function successCallback(response) {
return response.data;
}
}
})();<file_sep>package ir.saafta.model;
import javax.persistence.Entity;
import javax.persistence.Id;
import java.util.Date;
@Entity
public class DeletedUser {
@Id
private String username;
private Integer credit;
private Integer score;
private Date deletionDate;
private String deletedBy;
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public Integer getCredit() {
return credit;
}
public void setCredit(Integer credit) {
this.credit = credit;
}
public Integer getScore() {
return score;
}
public void setScore(Integer score) {
this.score = score;
}
public Date getDeletionDate() {
return deletionDate;
}
public void setDeletionDate(Date deletionDate) {
this.deletionDate = deletionDate;
}
public String getDeletedBy() {
return deletedBy;
}
public void setDeletedBy(String deletedBy) {
this.deletedBy = deletedBy;
}
}
<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('EventController', EventController);
/** @ngInject */
function EventController($log, $ngConfirm, $scope, $stateParams, NgTableParams, eventService, userService, toastr, host, $q) {
var vm = this;
vm.title = 'EventController';
vm.host = host;
activate();
vm.users = [];
////////////////
function activate() {
$log.debug('Event module activated');
$q.all([getUsers()]).then(function (data) {
data[0].forEach(function (user) {
vm.users.push({
id: user.username,
title: user.completeName
});
});
vm.eventTable = new NgTableParams({
page: 1,
count: 20
}, {
getData: getEventList
});
});
}
function getEventList(tabelparams) {
vm.loading = true;
var params = {
page: tabelparams.page() - 1,
size: tabelparams.count(),
sort: null
};
angular.forEach(tabelparams.sorting(), function (value, key) {
params.sort = key + ',' + value;
});
if (!params.sort) params.sort = 'time,desc';
var search = {
content: tabelparams.filter().content ? tabelparams.filter().content : null,
username: tabelparams.filter().user ? tabelparams.filter().user.id : null,
contentId: tabelparams.filter().contentId ? tabelparams.filter().contentId.id : null,
path: $stateParams.category ? $stateParams.category : null
};
return eventService.getEventList(params, search).then(function (data) {
tabelparams.total(data.totalElements);
vm.loading = false;
return data.content;
});
}
function getUsers() {
return userService.getAllUsers();
}
}
})();<file_sep>angular.module('appKitFormValidationDemo', [
'appKitCommon',
'appKit'
]).controller('AppKitFormValidationDemo', function ($scope) {
'use strict';
$scope.hobbyOptions = ['skiing', 'runing', 'eating', 'sleeping', 'reading', 'coding'];
$scope.hobbies = {};
$scope.noneOrAtLeastSelected = function (object, minCount) {
var count = 0;
minCount = minCount || 1;
count = object && Object.keys(object).filter(function (key) {
return !!object[key];
}).length;
return !(count === 0 || count >= minCount);
};
});
<file_sep>(function () {
'use strict';
angular
.module('app')
.factory('homeService', homeService);
/** @ngInject */
function homeService($log, $http, host) {
var base = host + 'home/';
var service = {
getPublishedBanners: getPublishedBanners,
getSuggestedApps: getSuggestedApps,
getNewApps: getNewApps,
getMostSeenApps: getMostSeenApps,
getBestApps: getBestApps
};
return service;
////////////////
function getPublishedBanners(params) {
return $http.get(host + "banner/published", {params: params}).then(successCallback);
}
function getSuggestedApps() {
return $http.get(host + "application/suggested").then(successCallback);
}
function getNewApps() {
return $http.get(host + "application/new").then(successCallback)
}
function getMostSeenApps() {
return $http.get(host + "application/mostSeen").then(successCallback);
}
function getBestApps() {
return $http.get(host + "application/best").then(successCallback);
}
function successCallback(response) {
return response.data;
}
}
})();<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('ApplicationController', ApplicationController);
/** @ngInject */
function ApplicationController($log, applicationService, host, $stateParams) {
var vm = this;
vm.title = 'ApplicationController';
activate();
vm.host = host;
vm.getStarClass = getStarClass;
vm.responsiveSetting = [
{
breakpoint: 964,
settings: {
slidesToShow: 2,
slidesToScroll: 2,
dots: true
}
},
{
breakpoint: 768,
settings: {
slidesToShow: 1,
slidesToScroll: 1,
dots: true
}
}
];
vm.slickConfig4 = {
method: {},
dots: true,
infinite: true,
speed: 300,
slidesToShow: 1,
centerMode: true,
variableWidth: true
};
vm.number4 = [{label: 225}, {label: 125}, {label: 200}, {label: 175}, {label: 150}, {label: 180}, {label: 300}, {label: 400}];
////////////////
function activate() {
$log.debug('Application module activated');
getApplication();
}
function getApplication() {
if ($stateParams.id)
applicationService.getApplication($stateParams.id).then(function (response) {
vm.application = response.data;
})
}
function getStarClass(app, index) {
if (app.rating - index > 1) {
return "star";
}
else if (app.rating - index > 0) {
return "star-half-o";
} else {
return "star-o";
}
}
}
})();<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('CreateUpdateBannerController', CreateUpdateBannerController);
/** @ngInject */
function CreateUpdateBannerController($log, $timeout, bannerService, $stateParams, toastr, $state,
$window, host) {
var vm = this;
vm.title = 'CreateUpdateBannerController';
vm.host = host;
vm.method = $stateParams.method;
vm.banner = {
id: $stateParams.id
};
vm.bounds = {
left: 0,
right: 20,
top: 0,
bottom: 20
};
vm.cropperWidth = $window.innerWidth * 0.4;
vm.save = save;
vm.reset = reset;
vm.getRatioDiff = getRatioDiff;
vm.date = new Date();
activate();
////////////////
function activate() {
$log.debug('Banner module activated');
getBanner();
}
function save() {
vm.loading = true;
switch (vm.method) {
case 'create':
bannerService.createBanner(vm.banner.label, vm.cropper.croppedImage)
.then(function (data) {
$timeout(function () {
vm.loading = false;
$state.go('root.banner');
}, 3000);
toastr.success('بنر با موفقیت افزوده شد.');
}, function () {
vm.loading = false;
toastr.error('خطا در ایجاد بنر!');
});
break;
case 'update':
var croppedImage = vm.cropper ? vm.cropper.croppedImage : null;
bannerService.updateBanner(vm.banner.id, vm.banner.name, croppedImage)
.then(function (data) {
vm.loading = false;
vm.banner = data;
toastr.success('بنر با موفقیت ویرایش شد.');
vm.date = new Date();
vm.cropper = null;
}, function () {
vm.loading = false;
toastr.error('خطا در ویرایش بنر!');
});
break;
}
}
function reset() {
switch (vm.method) {
case 'create':
vm.banner = {};
break;
case 'update':
getBanner();
}
}
function getBanner() {
if (vm.banner.id) {
vm.loading = true;
bannerService.getBanner(vm.banner.id).then(function (data) {
vm.loading = false;
vm.banner = data;
});
}
}
function getRatioDiff() {
return Math.abs((vm.bounds.right - vm.bounds.left) / (vm.bounds.top - vm.bounds.bottom) - (880 / 300));
}
}
})();<file_sep>(function () {
'use strict';
angular
.module('app')
.factory('ownerService', ownerService);
/** @ngInject */
function ownerService($log, $http, host) {
var base = host + 'owner/';
var service = {
getOwners: getOwners,
getAllOwners: getAllOwners,
getOwner: getOwner,
createOwner: createOwner,
updateOwner: updateOwner,
deleteOwner: deleteOwner
};
return service;
function successCallback(response) {
return response.data;
}
////////////////
function createOwner(owner) {
return $http.post(base, owner)
.then(successCallback);
}
function getOwners(params) {
return $http.get(base, {params: params})
.then(successCallback);
}
function getAllOwners() {
var url = base + 'all';
return $http.get(url)
.then(successCallback);
}
function getOwner(id) {
var url = base + id;
return $http.get(url)
.then(successCallback);
}
function updateOwner(owner) {
return $http.put(base, owner)
.then(successCallback);
}
function deleteOwner(id) {
var url = base + id;
return $http.delete(url)
.then(successCallback);
}
}
})();<file_sep>/**
* Created by reza on 11/14/16.
*/
(function () {
'use strict';
angular
.module('app')
.config(routeConfig);
/** @ngInject */
function routeConfig($stateProvider) {
$stateProvider
.state('root.banner', {
controller: 'BannerController',
controllerAs: 'vm',
data: {
roles: [
"admin"
]
},
resolve: {},
templateUrl: 'app/components/banner/list-banner.html',
url: '/banner'
})
.state('root.createUpdateBanner', {
controller: 'CreateUpdateBannerController',
controllerAs: 'vm',
data: {
roles: [
"admin"
]
},
resolve: {},
templateUrl: 'app/components/banner/create-update-banner.html',
url: '/banner/:method?id'
})
;
}
})();
<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('CommentController', CommentController);
/** @ngInject */
function CommentController($log, $ngConfirm, $scope, NgTableParams, commentService, userService, contentService, toastr, host, $q) {
var vm = this;
vm.title = 'CommentController';
vm.host = host;
vm.delete = Delete;
vm.approve = approve;
vm.reject = reject;
activate();
vm.users = [];
vm.contents = [];
////////////////
function activate() {
$log.debug('Comment module activated');
$q.all([getUsers(), getContents()]).then(function (data) {
data[0].forEach(function (user) {
vm.users.push({
id: user.username,
title: user.completeName
});
});
data[1].forEach(function (content) {
if (content.title && content.title !== "") {
console.log(content.title);
vm.contents.push({
id: content.id,
title: content.title
});
}
});
console.log(vm.users);
vm.commentTable = new NgTableParams({
page: 1,
count: 20
}, {
getData: getCommentList
});
});
}
function Delete(id) {
$ngConfirm({
title: 'حذف نظر',
content: '<span class="text-danger">{{"Are you sure about delete this item?"|translate}}</span>',
scope: $scope,
buttons: {
sayBoo: {
text: 'بله',
btnClass: 'btn-blue',
action: function (scope, button) {
vm.loading = true;
commentService.deleteComment(id)
.then(function () {
toastr.success('نظر با موفقیت حذف شد.');
vm.commentTable.reload();
vm.loading = false;
}, function () {
toastr.error('خطا در حذف نظر!');
vm.loading = false;
});
return true;
}
},
somethingElse: {
text: 'خیر',
btnClass: 'btn-orange',
action: function (scope, button) {
return true;
}
}
}
});
}
function getCommentList(tabelparams) {
// console.log(tabelparams.filter());
vm.loading = true;
var params = {
page: tabelparams.page() - 1,
size: tabelparams.count(),
sort: 'date,desc',
content: tabelparams.filter().content ? tabelparams.filter().content : null,
username: tabelparams.filter().user ? tabelparams.filter().user.id : null,
contentId: tabelparams.filter().contentId ? tabelparams.filter().contentId.id : null
};
return commentService.getCommentList(params).then(function (data) {
tabelparams.total(data.totalElements);
vm.loading = false;
return data.content;
});
}
function approve(comment) {
commentService.approveComment(comment.id).then(function () {
toastr.success('نظر با موفقیت تائید شد.');
vm.commentTable.reload();
}, function () {
toastr.error('خطا در تائید نظر!');
});
}
function reject(comment) {
commentService.rejectComment(comment.id).then(function () {
toastr.success('نظر با موفقیت رد شد.');
vm.commentTable.reload();
}, function () {
toastr.error('خطا در رد نظر!');
});
}
function getUsers() {
return userService.getAllUsers();
}
function getContents() {
return contentService.getAllContents();
}
}
})();<file_sep>(function () {
'use strict';
angular
.module('app')
.directive('commandButtons', commandButtons);
/** @ngInject */
function commandButtons() {
var directive = {
bindToController: true,
controller: ButtonsController,
controllerAs: 'vm',
restrict: 'E',
scope: {
cancel: '&?',
delete: '&?',
disabled: '=?',
edit: '&?',
toggle: '=?'
},
templateUrl: 'app/directives/ng-table/command-buttons.html'
};
return directive;
}
/** @ngInject */
function ButtonsController() {
/* jshint validthis: true */
var vm = this;
vm.toggled = toggled;
function toggled() {
vm.toggle = !vm.toggle;
}
}
})();<file_sep>package ir.saafta.repo;
import ir.saafta.model.Category;
import org.springframework.data.repository.PagingAndSortingRepository;
/**
* Created by reza on 11/12/16.
*/
public interface CategoryRepository extends PagingAndSortingRepository<Category, Long> {
}
<file_sep>package ir.saafta.conf;
import ir.saafta.service.LoginAttemptService;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationListener;
import org.springframework.security.authentication.event.AuthenticationFailureBadCredentialsEvent;
import org.springframework.security.web.authentication.WebAuthenticationDetails;
import org.springframework.stereotype.Component;
@Component
public class AuthenticationFailureListener
implements ApplicationListener<AuthenticationFailureBadCredentialsEvent> {
private final Logger logger = LoggerFactory.getLogger(this.getClass());
@Autowired
private LoginAttemptService loginAttemptService;
public void onApplicationEvent(AuthenticationFailureBadCredentialsEvent e) {
WebAuthenticationDetails auth = (WebAuthenticationDetails)
e.getAuthentication().getDetails();
logger.info("AuthenticationFailureListener " + auth.getRemoteAddress());
}
}<file_sep>(function () {
'use strict';
angular
.module('app')
.directive('appKitMenu', appKitMenu);
/** @ngInject */
function appKitMenu() {
'use strict';
return {
restrict: 'AE',
link: function (scope, element) {
$(element).metisMenu();
}
};
}
})();
<file_sep>package ir.saafta.web;
import com.sun.jersey.core.impl.provider.entity.XMLJAXBElementProvider;
import ir.saafta.dto.ApplicationStatus;
import ir.saafta.exception.HttpUnauthorizedException;
import ir.saafta.model.Application;
import ir.saafta.model.Transaction;
import ir.saafta.model.User;
import ir.saafta.model.UserApp;
import ir.saafta.repo.ApplicationRepository;
import ir.saafta.repo.TransactionRepository;
import ir.saafta.repo.UserAppRepository;
import ir.saafta.service.MultipartFileSender;
import ir.saafta.service.UserDetailManager;
import org.apache.catalina.servlet4preview.http.HttpServletRequest;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.access.AccessDeniedException;
import org.springframework.security.core.Authentication;
import org.springframework.web.bind.annotation.*;
import javax.servlet.http.HttpServletResponse;
import java.util.Arrays;
import java.util.Date;
/**
* Created by Reza on 1/20/2017.
*/
@RestController
@RequestMapping("/download")
public class DownloadController {
private final String simorghPackage = "ir.simorghmarket.simorgh";
private final Logger logger = LoggerFactory.getLogger(this.getClass());
private ApplicationRepository applicationRepository;
private UserDetailManager userDetailManager;
private UserAppRepository userAppRepository;
private TransactionRepository transactionRepository;
@Autowired
public DownloadController(ApplicationRepository applicationRepository, UserDetailManager userDetailManager,
UserAppRepository userAppRepository, TransactionRepository transactionRepository) {
this.applicationRepository = applicationRepository;
this.userDetailManager = userDetailManager;
this.userAppRepository = userAppRepository;
this.transactionRepository = transactionRepository;
}
@ResponseBody
@RequestMapping(value = "/{appId}/*.apk", method = RequestMethod.GET)
public void install(@PathVariable Long appId, String phoneNumber, Authentication authentication,
HttpServletRequest request, HttpServletResponse response) throws Exception {
Application application = applicationRepository.findOne(appId);
logger.info("Download application with id " + application.getId());
logger.info("\tFile id is " + application.getLastFile().getId());
if(application.getFee() == null)
application.setFee(0);
// if this is an anonymous user and app is free
if(authentication == null && application.getFee() == 0) {
// check if it comes from application
if (request.getHeader("User-Agent").startsWith("app_") || request.getHeader("User-Agent").startsWith("safeHome_")) {
logger.info("Installing app anonymously with id " + application.getId());
UserApp userApp = userAppRepository.findBySessionAndContent(request.getHeader("User-Agent"), application);
if (userApp == null) {
userApp = new UserApp();
userApp.setContent(application);
userApp.setSession(request.getHeader("User-Agent"));
userApp.setInstallDate(new Date());
application.setInstallationCount(application.getInstallationCount() == null ? 1 : application.getInstallationCount() + 1);
userAppRepository.save(userApp);
applicationRepository.save(application);
logger.info("free ( " + request.getHeader("User-Agent") + " ): Application installation count updated to " + application.getInstallationCount());
}
MultipartFileSender.fromFile(application.getLastFile()).with(request).with(response).serveResource();
}
return;
} else if (authentication == null) { // if app is not free and user ins anonymous raise an exception
throw new HttpUnauthorizedException();
}
String username = authentication.getName();
User user = (User) userDetailManager.loadUserByUsername(username);
if(user.getCredit() == null)
user.setCredit(0);
logger.info("username: " + username + ", application fee: " + application.getFee());
UserApp userApp = userAppRepository.findByUserAndContent(user, application);
// if user in just installing the application
if (userApp == null || userApp.getInstallDate() == null) {
logger.info("First time download");
// if user has enough credit to get the app
if (user.getCredit() >= application.getFee()) {
// register a transaction
Transaction transaction = new Transaction(new Date(), application.getFee(),
"buy", null, application, username);
transaction = transactionRepository.save(transaction);
logger.info("Transaction saved( user: " + transaction.getUsername() +
", appId:" + transaction.getApplication().getId() +
", type:" + transaction.getType() +
", amount:" + transaction.getAmount() +
", id:" + transaction.getId() +
" @" + transaction.getDate()
);
// update user credit
logger.info("Update user credit from " + user.getCredit());
user.setCredit(user.getCredit() - application.getFee());
user = userDetailManager.save(user);
logger.info("Update user credit to " + user.getCredit());
// register a new user-application object or update existing one
if (userApp == null) {
userApp = new UserApp(user, application, new Date(), phoneNumber);
}
else
userApp.setInstallDate(new Date());
userApp.setBought(application.getFee() != null && application.getFee() > 0);
// now increment installation count of the application as it is getting installed by the user
int installationCount = application.getInstallationCount() == null ? 1 : application.getInstallationCount() + 1;
application.setInstallationCount(installationCount);
application = applicationRepository.save(application);
logger.info("Application installation count updated to " + application.getInstallationCount());
} else {
logger.info("User has not enough credit to buy the app");
throw new AccessDeniedException("You should be logged in to download non-free applications");
}
}
// update the version that user has installed in the storage
userApp.setCurrentVersion(application.getLastFile().getVersion());
userApp = userAppRepository.save(userApp);
logger.info("UserApp (id: " + userApp.getId() + ")");
if (request.getHeader("User-Agent").startsWith("app_") || request.getHeader("User-Agent").startsWith("safeHome_"))
MultipartFileSender.fromFile(application.getLastFile()).with(request).with(response).serveResource();
}
@ResponseBody
@RequestMapping(value = "/simorgh/*.apk", method = RequestMethod.GET)
public void installSimorgh(HttpServletResponse response, HttpServletRequest request) throws Exception {
ApplicationStatus status = request.getHeader("User-Agent").startsWith("safeHome_") ?
ApplicationStatus.LAUNCHER : ApplicationStatus.NON_LAUNCHER;
Application simorgh = applicationRepository.findByAppPackageAndStatus(simorghPackage, status);
if (simorgh == null || simorgh.getLastFile() == null)
return;
MultipartFileSender.fromFile(simorgh.getLastFile()).with(request).with(response).serveResource();
}
@ResponseBody
@RequestMapping(value = "/{appId}", method = RequestMethod.DELETE)
public void uninstall(@PathVariable Long appId, String username, String phoneNumber) {
User user = (User) userDetailManager.loadUserByUsername(username);
Application application = applicationRepository.findOne(appId);
UserApp userApp = userAppRepository.findByUserAndContent(user, application);
if (userApp != null) {
userApp.setUninstallDate(new Date());
userAppRepository.save(userApp);
}
}
}
<file_sep>package ir.saafta.repo;
import ir.saafta.model.Application;
import ir.saafta.model.Content;
import ir.saafta.model.User;
import ir.saafta.model.UserApp;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.data.repository.PagingAndSortingRepository;
/**
* Created by Reza on 1/20/2017.
*/
public interface UserAppRepository extends PagingAndSortingRepository<UserApp, Long> {
UserApp findByUserAndContent(User user, Content content);
UserApp findByPhoneNumberAndContent(String phoneNumber, Content content);
UserApp findBySessionAndContent(String session, Content content);
Page<UserApp> findByUser(Pageable pageable, User user);
Page<UserApp> findByUserAndBookmarked(Pageable pageable, User user, Boolean bookmarked);
}
<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('CreateUpdateUserController', CreateUpdateUserController);
/** @ngInject */
function CreateUpdateUserController($log, $ngConfirm, toastr, userService, userGroupService, $stateParams, host, util,
$scope, $timeout, $state, principal) {
var vm = this;
vm.title = 'CreateUpdateUserController';
vm.tmpData = new Date(); // to display image content after change
vm.host = host;
vm.method = $stateParams.method;
vm.user = {
username: $stateParams.username,
authority: null
};
vm.errors = [];
vm.save = save;
vm.reset = reset;
vm.resetPassword = <PASSWORD>;
vm.addCredit = addCredit;
vm.reduceCredit = reduceCredit;
vm.saveProfilePicture = saveProfilePicture;
vm.deleteProfilePicture = deleteProfilePicture;
vm.checkUsername = checkUsername;
vm.checkEmail = checkEmail;
vm.checkMobilePhone = checkMobilePhone;
vm.getError = getError;
vm.checkField = checkField;
vm.checkErrors = checkErrors;
vm.addGroup = addGroup;
vm.removeGroup = removeGroup;
vm.isAdmin = false;
activate();
////////////////
function activate() {
$log.debug('User module activated', $stateParams.username);
getUserGroups();
principal.identity().then(function (currentUser) {
vm.me = currentUser.username === vm.user.username;
currentUser.authorities.forEach(function (auth) {
if (auth.authority === "admin") {
vm.isAdmin = true;
}
});
if (vm.method === 'update') {
if (vm.isAdmin && vm.user.username !== 'me') {
getAuthorities().then(getUser);
}
else if (vm.user.username === 'me') {
getMe();
vm.me = true;
}
} else {
getAuthorities().then(function (data) {
vm.userAuthority = data.filter(function (authority) {
return authority.authority === 'user';
})[0];
});
}
});
}
function checkForm(form) {
if(!form || form.$valid)
return;
if (form.$error.unique) {
form.$error.unique.forEach(function (item) {
vm.errors.push({
name: item.$name,
caption: item.$name + ' is redundant'
})
})
}
if(form.$error.pattern) {
form.$error.pattern.forEach(function (item) {
vm.errors.push({
name: item.$name,
caption: item.$name + ' does not have valid value'
})
})
}
if(form.$error.required) {
form.$error.required.forEach(function (item) {
vm.errors.push({
name: item.$name,
caption: item.$name + ' is required'
})
})
}
}
function checkErrors(userForm) {
vm.errors = [];
// console.log('userForm',userForm);
// console.log('userInfoForm', userInfoForm);
if(vm.user.password && vm.user.password !== <PASSWORD>)
vm.errors.push({
name: 'checkPassword',
caption: 'Password does not match entered amount'
});
checkForm(userForm);
// checkForm(userInfoForm);
}
function save(userForm) {
checkErrors(userForm);
if(vm.errors.length > 0)
return;
vm.user.authorities = [];
vm.user.authorities.push({
authority: vm.userAuthority.authority,
username: vm.user.username
});
vm.loading = true;
switch (vm.method) {
case 'create':
userService.createUser(vm.user).then(function () {
toastr.success('کاربر با موفقیت ایجاد شد.');
$timeout(function () {
vm.loading = false;
$state.go('root.createUpdateUser', {'method':'update', 'username':vm.user.username});
}, 3000);
}, function (error) {
vm.loading = false;
toastr.error('خطا در ایجاد کاربر!');
});
break;
case 'update':
userService.updateUser(vm.user).then(function () {
vm.loading = false;
toastr.success('کابر با موفقیت به روزرسانی شد.');
}, function () {
vm.loading = false;
toastr.error('خطا در به روزرسانی کاربر!');
});
break;
default:
vm.loading = false;
}
}
function getAuthorities() {
return userService.getAuthorities().then(function (data) {
return vm.authorities = data;
});
}
function getCaption(authority) {
for (var i = 0; i < vm.authorities.length; i++) {
if (vm.authorities[i].authority === authority)
return vm.authorities[i].caption;
}
}
function reset(form) {
vm.errors = [];
switch (vm.method) {
case 'create':
if(form) {
angular.forEach(form, function (value, key) {
if(key.indexOf('$') < 0){
value.$$rawModelValue = null;
value.$$lastCommittedViewValue = null;
value.$$rawModelValue = null;
value.$viewValue = null;
value.$error = {};
value.$render();
console.log(key, value);
}
});
form.$error = {};
}
vm.user = {
authorities: []
};
break;
case 'update':
getUser();
}
}
function getUser() {
vm.userAuthorities = [];
if (vm.user.username) {
vm.loading = true;
userService.getUser(vm.user.username).then(function (data) {
vm.user = data;
// console.log(data);
vm.passwordCheck = <PASSWORD>;
vm.user.authorities.forEach(function (authority) {
vm.userAuthority = {
"authority": authority.authority,
"caption": getCaption(authority.authority)
};
});
vm.loading = false;
}, function () {
vm.loading = false;
});
}
}
function getMe() {
vm.loading = true;
return userService.getMe().then(function (data) {
vm.user = data;
vm.loading = false;
}, function () {
vm.loading = false;
});
}
function resetPassword() {
vm.loading = true;
if (vm.isAdmin) {
userService.resetPassword(vm.user.username, vm.user.password).then(function () {
vm.loading = false;
toastr.success('کلمه عبور با موفقیت به روزرسانی شد.');
}, function () {
vm.loading = false;
toastr.error('خطا در به روزرسانی کلمه عبور!');
});
} else {
userService.updatePassword(vm.user.username, vm.user.password, vm.user.oldPassword).then(function () {
vm.loading = false;
toastr.success('کلمه عبور با موفقیت به روزرسانی شد.');
}, function () {
vm.loading = false;
toastr.error('خطا در به روزرسانی کلمه عبور!');
});
}
}
function addCredit() {
userService.addCredit(vm.user.username, vm.creditToAdd, vm.creditComment).then(function (data) {
toastr.success('اعتبار با موفقیت افزوده شد.');
vm.user.credit = data;
vm.creditToAdd = null;
vm.creditComment = null;
}, function () {
toastr.error('خطا در افزایش موجودی!');
});
}
function deleteProfilePicture() {
$ngConfirm({
title: 'حذف تصویر کاربری',
content: '<span class="text-danger">{{"Are you sure about delete this item?"|translate}}</span>',
scope: $scope,
buttons: {
sayBoo: {
text: 'بله',
btnClass: 'btn-blue',
action: function(scope, button){
vm.loading = true;
userService.deleteProfilePicture(vm.user.username).then(function () {
util.toast('success','تصویر با موفقیت حذف شد.');
vm.user.profilePicture = null;
vm.loading = false;
}, function () {
util.toast('error','خطا در حذف تصویر!');
vm.loading = false;
});
return true;
}
},
somethingElse: {
text: 'خیر',
btnClass: 'btn-orange',
action: function(scope, button){
return true;
}
}
}
});
}
function reduceCredit() {
userService.addCredit(vm.user.username, vm.creditToAdd * -1, vm.creditComment).then(function (data) {
toastr.success('اعتبار با موفقیت کسر شد.');
vm.user.credit = data;
vm.creditToAdd = null;
vm.creditComment = null;
}, function () {
toastr.error('خطا در کاهش موجودی!');
});
}
function saveProfilePicture(dataUrl) {
vm.loading = true;
userService.saveProfilePicture(vm.user.username, dataUrl)
.then(function (response) {
vm.user.profilePicture = response;
vm.tmpData = new Date();
util.toast('success', 'تصویر کاربری با موفقیت به روزرسانی شد.');
vm.cropper = {};
vm.cropper.sourceImage = null;
vm.cropper.croppedImage = null;
vm.loading = false;
}, function (error) {
if (error.status > 0) {
vm.errorMsg = error.status + ': ' + error.data;
}
util.toast('error', 'خطا در بارگذاری تصویر!');
vm.loading = false;
});
}
function getUserGroups() {
userGroupService.getAllUserGroups().then(function (data) {
vm.groups = data;
})
}
function checkUsername(form) {
vm.loading = true;
userService.usernameIsValid(vm.user.username).then(function (isValid) {
form.username.$setValidity('unique', isValid);
vm.loading = false;
}, function (error) {
vm.loading = false;
});
}
function checkEmail(form) {
// console.log('checkEmail');
vm.loading = true;
return userService.emailIsValid(vm.user.email, vm.user.username).then(function (isValid) {
form.email.$setValidity('unique', isValid);
vm.loading = false;
checkErrors(form);
}, function (error) {
vm.loading = false;
});
}
function checkMobilePhone(form) {
vm.loading = true;
userService.mobilePhoneIsValid(vm.user.mobilePhone, vm.user.username).then(function (isValid) {
form.mobilePhone.$setValidity('unique', isValid);
vm.loading = false;
checkErrors(form);
}, function (error) {
vm.loading = false;
});
}
function getError(name) {
// console.log('getError');
var res = null;
for(var i = 0; i < vm.errors.length; i++) {
if(vm.errors[i].name === name)
res = vm.errors[i];
}
return res;
}
function checkField(field) {
// console.log('checkField', field, getError(field.$name));
if(field.$name === 'email')
checkEmail(field).then(function () {
check();
});
else
check();
function check() {
if(!field || field.$valid || getError(field.$name))
return;
if (field.$error.unique) {
vm.errors.push({
name: field.$name,
caption: field.$name + ' is redundant'
})
}
if(field.$error.pattern) {
vm.errors.push({
name: field.$name,
caption: field.$name + ' does not have valid value'
})
}
if(field.$error.required) {
vm.errors.push({
name: field.$name,
caption: field.$name + ' is required'
})
}
}
}
function addGroup() {
userService.addGroup(vm.user.username, vm.group.id).then(function (success) {
vm.user.userGroups.push(angular.copy(vm.group));
util.toast('success', 'گروه با موفقیت افزوده شد.');
}, function (error) {
util.toast('error', 'خطا در افزودن گروه به کاربر');
})
}
function removeGroup(group) {
userService.removeGroup(vm.user.username, group.id).then(function (success) {
if (!vm.user.userGroups)
vm.user.userGroups = [];
var i = 0;
for (i; i < vm.user.userGroups.length; i++) {
if (vm.user.userGroups[i].id === group.id)
break;
}
vm.user.userGroups.splice(i, 1);
util.toast('success', 'گروه با موفقیت حذف شد.');
}, function (error) {
util.toast('error', 'خطا در حذف گروه از کاربر');
})
}
var stillClearPassword = vm.user.password !== undefined && vm.user.password !== null;
$scope.$watch(angular.bind(this, function () {
return this.user.password;
}), function (newVal) {
if (stillClearPassword && newVal !== undefined && newVal !== null) {
vm.user.password = '';
stillClearPassword = false;
}
});
}
})();<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('UserController', UserController);
/** @ngInject */
function UserController($log, NgTableParams, userService) {
var vm = this;
vm.title = 'UserController';
vm.userTable = new NgTableParams({
page: 0,
count: 20
}, {
getData: getUsers
});
activate();
////////////////
function activate() {
$log.debug('User module activated');
}
function getUsers(tabelparams) {
var params = {
page: tabelparams.page(),
size: tabelparams.count()
};
return userService.getUsers(params).then(function (data) {
tabelparams.total(data.totalElemets);
return data.content;
});
}
}
})();<file_sep>(function () {
'use strict';
angular
.module('app')
.directive('appKitModule', appKitModule);
/** @ngInject */
function appKitModule() {
'use strict';
return {
restrict: "AE",
transclude: {
'more': '?moreMenu',
'body': 'paneBody',
'footer': '?paneFooter'
},
replace: true,
require: '?^^appKitMasonry',
templateUrl: 'app/directives/appKitModule/appKitModule.html',
scope: {
wrapperClass: '@',
moduleClass: '@',
contentClass: '@',
title: '@',
meta: '@',
controls: "=?",
collapsed: '@'
},
link: function (scope, element, attrs, appKitMasonry, transclude) {
if (typeof scope.controls === 'undefined') {
scope.controls = !!appKitMasonry;
}
if (typeof scope.collapsed === 'undefined') {
scope.collapsed = false;
}
scope.showFooter = transclude.isSlotFilled('footer');
scope.showMore = transclude.isSlotFilled('more');
if (typeof scope.wrapperClass === 'undefined') {
if (scope.title || scope.meta) {
scope.wrapperClass = 'module-headings';
} else {
scope.wrapperClass = 'module-headings';
}
}
if (appKitMasonry) {
appKitMasonry.layout();
}
scope.closeModule = function (ev) {
if (appKitMasonry) {
appKitMasonry.closeModule($(ev.target).closest(appKitMasonry.selector));
} else {
$(element).remove();
}
};
scope.collapseModule = function (ev) {
scope.collapsed = !scope.collapsed;
};
}
};
}
})();
<file_sep>package ir.saafta.repo;
import ir.saafta.dto.SessionStatus;
import ir.saafta.model.Session;
import ir.saafta.model.SpringSession;
import org.springframework.data.repository.PagingAndSortingRepository;
import java.util.ArrayList;
/**
* Created by rkesh on 6/30/2017.
*/
public interface SpringSessionRepository extends PagingAndSortingRepository<SpringSession, String> {
ArrayList<SpringSession> findAllByPrincipalNameIsNotNull();
}
<file_sep>/**
* Created by Reza on 11/19/2016.
*/
(function () {
'use strict';
angular
.module('app')
.controller('CreateUpdateFileController', CreateUpdateFileController);
/** @ngInject */
function CreateUpdateFileController(host, file, application, $timeout, Upload) {
var vm = this;
vm.application = application;
vm.file = file | {};
vm.uploadFile = uploadFile;
function uploadFile(file) {
file.upload = Upload.upload({
url: host + '/application/4/file',
data:{username: 'reza', file: file}
});
file.upload.then(function (response) {
$timeout(function () {
file.result = response.data;
});
},
function (response) {
if (response.status > 0)
$scope.errorMsg = response.status + ': ' + response.data;
},
function (evt) {
// Math.min is to fix IE which reports 200% sometimes
file.progress = Math.min(100, parseInt(100.0 * evt.loaded / evt.total));
});
}
}
});
<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('CreateUpdateAdController', CreateUpdateAdController);
/** @ngInject */
function CreateUpdateAdController($log, $timeout, adService, userService, $stateParams, toastr,
$state, $filter, host, $window) {
var vm = this;
vm.title = 'CreateUpdateAdController';
vm.method = $stateParams.method;
vm.ad = {
id: $stateParams.id
};
vm.bounds = {
left: 0,
right: 20,
top: 0,
bottom: 20
};
vm.cropperWidth = $window.innerWidth * 0.4;
vm.host = host;
vm.save = save;
vm.reset = reset;
vm.date = new Date();
activate();
////////////////
function activate() {
$log.debug('Ad module activated');
getAd();
getUsers();
}
function save() {
var ad = angular.copy(vm.ad);
ad.fromDate = $filter('sDate')(vm.ad.fromDate);
ad.toDate = $filter('sDate')(vm.ad.toDate);
ad.username = vm.ad.user.username;
console.log('save');
vm.loading = true;
switch (vm.method) {
case 'create':
adService.createAd(ad, vm.cropper.croppedImage)
.then(function (data) {
$timeout(function () {
$state.go('root.ad');
vm.loading = false;
}, 1000);
toastr.success('تبلیغات با موفقیت افزوده شد.');
}, function () {
vm.loading = false;
toastr.error('خطا در ایجاد تبلیغات!');
});
break;
case 'update':
console.log('save ', vm.ad);
var croppedImage = vm.cropper ? vm.cropper.croppedImage : null;
adService.updateAd(vm.ad.id, ad, croppedImage)
.then(function (response) {
getAd(response.data);
toastr.success('تبلیغات با موفقیت ویرایش شد.');
vm.date = new Date();
vm.cropper = null;
vm.loading = false;
}, function () {
vm.loading = false;
toastr.error('خطا در ویرایش تبلیغات!');
});
break;
}
}
function reset() {
switch (vm.method) {
case 'create':
vm.ad = {};
break;
case 'update':
getAd();
}
}
function getAd(ad) {
if (ad) {
loadAd(ad);
}
else if (vm.ad.id) {
vm.loading = true;
adService.getAd(vm.ad.id).then(function (data) {
loadAd(data);
vm.loading = false;
}, function () {
util.toast('error', 'APP.MESSAGES.FAILURE');
vm.loading = false;
});
}
function loadAd(advertisement) {
vm.ad = advertisement;
vm.ad.label = advertisement.picture.label;
}
}
function getUsers() {
userService.getAllUsers().then(function (data) {
vm.users = data;
}, function () {
toastr.error('خطا در بارگذاری لیست کاربران!')
});
}
}
})();<file_sep>package ir.saafta.conf;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.security.core.AuthenticationException;
import org.springframework.security.web.AuthenticationEntryPoint;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
/**
* Created by reza on 11/12/16.
*/
public class RestAuthenticationEntryPoint implements AuthenticationEntryPoint {
private final Logger logger = LoggerFactory.getLogger(this.getClass());
@Override
public void commence(HttpServletRequest request, HttpServletResponse response, AuthenticationException authException) throws IOException, ServletException {
response.sendError(HttpServletResponse.SC_UNAUTHORIZED);
logger.info("RestAuthenticationEntryPoint");
}
}
<file_sep>(function () {
'use strict';
angular
.module('app')
.directive('appKitResponsive', appKitResponsive);
/** @ngInject */
function appKitResponsive($window) {
'use strict';
return {
restrict: 'AE',
link: function (scope, element) {
$window = angular.element($window);
function adaptToScreenSize() {
scope.compact = $window.width() <= 1200;
scope.mobile = $window.width() <= 768;
}
scope.navOpen = false;
scope.showFooter = true;
$window.bind('resize', function () {
scope.$apply(adaptToScreenSize);
});
$window.bind('load', function () {
$(element).removeClass('preload');
});
adaptToScreenSize();
}
};
}
})();
<file_sep>CREATE TABLE `user_content` (
`id` BIGINT(20) NOT NULL AUTO_INCREMENT,
`username` VARCHAR(50),
`content_id` BIGINT(20),
`phone_number` VARCHAR(14),
`rating` DOUBLE DEFAULT 0,
PRIMARY KEY (`id`)
);
INSERT INTO `user_content` (
`id`,
`username`,
`content_id`,
`phone_number`,
`rating`
) SELECT
id,
username,
app_id,
phone_number,
rating
FROM user_app;
ALTER TABLE `user_app`
DROP COLUMN `username`,
DROP COLUMN `app_id`,
DROP COLUMN `phone_number`,
DROP INDEX `user_app_fk1_username`,
DROP INDEX `user_app_fk2_app_id`,
DROP FOREIGN KEY `user_app_fk1_username`,
DROP FOREIGN KEY `user_app_fk2_app_id`;
ALTER TABLE `user_app`
CHANGE `id` `id` BIGINT(20) UNSIGNED NOT NULL,
DROP PRIMARY KEY;
ALTER TABLE `user_content`
CHANGE `id` `id` BIGINT(20) UNSIGNED NOT NULL AUTO_INCREMENT;
ALTER TABLE `user_app`
ADD CONSTRAINT `user_app_user_content_id_fk` FOREIGN KEY (`id`) REFERENCES `user_content` (`id`)
ON UPDATE CASCADE
ON DELETE CASCADE;
ALTER TABLE `user_content`
CHANGE `content_id` `content_id` BIGINT(20) UNSIGNED NULL,
ADD CONSTRAINT `user_content_user_username_fk` FOREIGN KEY (`username`) REFERENCES `user` (`username`)
ON UPDATE CASCADE
ON DELETE CASCADE,
ADD CONSTRAINT `user_content_content_id_fk` FOREIGN KEY (`content_id`) REFERENCES `content` (`id`)
ON UPDATE CASCADE
ON DELETE CASCADE;
;
ALTER TABLE `feedback`
CHANGE `rate` `rate` DOUBLE DEFAULT 0 NULL;
ALTER TABLE `user_content`
CHANGE `rating` `rating` DOUBLE DEFAULT -1 NULL;
ALTER TABLE `feedback`
CHANGE `liked` `liked` TINYINT(1) DEFAULT 0 NULL,
CHANGE `disliked` `disliked` TINYINT(1) DEFAULT 0 NULL;
ALTER TABLE `application`
ADD COLUMN `recently_updated` BINARY(1) DEFAULT '0' NULL
AFTER `last_file_id`;
ALTER TABLE `advertisement`
ADD COLUMN `seen_count` BINARY(1) DEFAULT '0' NULL
AFTER `toDate`;
ALTER TABLE `advertisement`
CHANGE `fromDate` `from_date` DATETIME NULL,
CHANGE `toDate` `to_date` DATETIME NULL;
ALTER TABLE `news`
DROP COLUMN `content_id`,
CHANGE `context` `body` TEXT CHARSET utf8
COLLATE utf8_general_ci NULL,
ADD COLUMN `writer` VARCHAR(60) NULL
AFTER `date`,
ADD COLUMN `lead` VARCHAR(200) NULL
AFTER `writer`;
ALTER TABLE `user_app`
ADD COLUMN `bookmarked` BINARY(1) DEFAULT '0' NULL AFTER `current_version`;
ALTER TABLE `application`
ADD COLUMN `installation_count` INT(11) DEFAULT 0 NULL
AFTER `recently_updated`;
ALTER TABLE `application`
ADD COLUMN `in_app_payment` BINARY(1) DEFAULT '0' NULL
AFTER `installation_count`;
ALTER TABLE `file`
ADD COLUMN `minimum_android_version` VARCHAR(255) NULL AFTER `version`;
ALTER TABLE `access_file`
CHANGE `id` `id` BIGINT(20) UNSIGNED NOT NULL AUTO_INCREMENT;
ALTER TABLE `access_file`
ADD UNIQUE INDEX `Unique_file_access` (`access_id`, `file_id`);
ALTER TABLE `application`
ADD COLUMN `suggestion_priority` BIGINT(20) UNSIGNED NULL AFTER `in_app_payment`;
CREATE TABLE `suggestion_priority` (
`id` BIGINT(20) UNSIGNED NOT NULL AUTO_INCREMENT,
`app_id` BIGINT(20) UNSIGNED,
`priority` INT(2) UNSIGNED DEFAULT 0,
PRIMARY KEY (`id`),
CONSTRAINT `suggestion_priority_application` FOREIGN KEY (`app_id`) REFERENCES `application` (`id`)
ON UPDATE CASCADE
ON DELETE CASCADE
);
ALTER TABLE `application`
ADD COLUMN `suggested_app` BIGINT(1) DEFAULT 0 NULL AFTER `suggestion_priority`;
CREATE TABLE `event` (
`id` BIGINT(20) UNSIGNED NOT NULL AUTO_INCREMENT,
`time` DATETIME NOT NULL,
`action` VARCHAR(50) NOT NULL,
`username` VARCHAR(50),
`remote_addr` VARCHAR(30),
`host` VARCHAR(50),
`session_id` VARCHAR(100),
`Cookie` VARCHAR(200),
`method` VARCHAR(10),
`params` TEXT,
`body` TEXT,
PRIMARY KEY (`id`),
CONSTRAINT `fk_event_user_username` FOREIGN KEY (`username`) REFERENCES `user` (`username`)
ON UPDATE CASCADE
ON DELETE NO ACTION
);
ALTER TABLE `event`
ADD COLUMN `user-agent` TEXT NULL AFTER `body`;
ALTER TABLE `event`
ADD COLUMN `path` VARCHAR(50) NULL AFTER `user-agent`;
ALTER TABLE `event`
CHANGE `user-agent` `user_agent` TEXT CHARSET utf8 COLLATE utf8_general_ci NULL;
ALTER TABLE `application`
ADD CONSTRAINT `foreign_key_application_file_last_file` FOREIGN KEY (`last_file_id`) REFERENCES `file` (`id`)
ON UPDATE CASCADE
ON DELETE NO ACTION;
SET FOREIGN_KEY_CHECKS = 0;
ALTER TABLE `user`
CHANGE `username` `username` VARCHAR(50)
CHARSET utf8
COLLATE utf8_bin NOT NULL;
SET FOREIGN_KEY_CHECKS = 1;
CREATE TABLE `session` (
`session_id` VARCHAR(100) NOT NULL,
`username` VARCHAR(50) NOT NULL,
`creation_time` DATETIME,
`finish_time` DATETIME,
`status` INT(1),
`last_request` TIMESTAMP,
`remote_addr` VARCHAR(30),
`device_info` VARCHAR(255),
PRIMARY KEY (`session_id`)
);
ALTER TABLE `user_content`
ADD COLUMN `install_date` DATETIME NULL
AFTER `rating`,
ADD COLUMN `uninstall_date` DATETIME NULL
AFTER `install_date`,
ADD COLUMN `current_version` VARCHAR(255) NULL
AFTER `uninstall_date`,
ADD COLUMN `bookmarked` BINARY(1) NULL
AFTER `current_version`;
ALTER TABLE `company`
ADD COLUMN `email` VARCHAR(50) NULL
AFTER `url`,
ADD COLUMN `phone_number` VARCHAR(15) NULL
AFTER `email`,
ADD COLUMN `type` INT(1) NULL
AFTER `phone_number`;
RENAME TABLE
`company` TO `owner`;
ALTER TABLE `application`
CHANGE `company_id` `owner_id` BIGINT(20) UNSIGNED NULL;
ALTER TABLE `application`
DROP INDEX `foreign_key_application_company`;
ALTER TABLE `application`
ADD CONSTRAINT `foreign_key_application_owner` FOREIGN KEY (`owner_id`) REFERENCES `owner` (`id`)
ON UPDATE CASCADE
ON DELETE NO ACTION;
ALTER TABLE `comment`
ADD COLUMN `approved` BINARY DEFAULT '0' NULL
AFTER `user_id`;
ALTER TABLE `application`
CHANGE `app_package` `app_package` VARCHAR(255)
CHARSET utf8
COLLATE utf8_general_ci NOT NULL,
CHANGE `name` `name` VARCHAR(255)
CHARSET utf8
COLLATE utf8_general_ci NOT NULL;
CREATE TABLE `user_group` (
`id` BIGINT(20) UNSIGNED NOT NULL AUTO_INCREMENT,
`title` VARCHAR(50) NOT NULL,
`logo_id` BIGINT(20) UNSIGNED,
PRIMARY KEY (`id`)
);
ALTER TABLE `user`
ADD COLUMN `user_group_id` BIGINT(20) UNSIGNED NULL
AFTER `imei`;
ALTER TABLE `user`
ADD CONSTRAINT `user_fk2_group` FOREIGN KEY (`user_group_id`) REFERENCES `user_group` (`id`)
ON UPDATE CASCADE
ON DELETE NO ACTION;
ALTER TABLE `application`
DROP FOREIGN KEY `foreign_key_application_category`;
ALTER TABLE `application`
ADD CONSTRAINT `foreign_key_application_category` FOREIGN KEY (`category_id`) REFERENCES `category` (`id`)
ON UPDATE CASCADE
ON DELETE RESTRICT;
ALTER TABLE `application`
CHANGE `category_id` `category_id` BIGINT(20) UNSIGNED NOT NULL;
ALTER TABLE `user`
ADD UNIQUE INDEX `user_email_unique` (`email`),
ADD UNIQUE INDEX `user_phoneNumber_unique` (`mobile_phone`);
ALTER TABLE `user_group`
ADD UNIQUE INDEX `user_group_name_unique` (`title`);
ALTER TABLE `category`
ADD UNIQUE INDEX `category_name_unique` (`name`);
ALTER TABLE `file`
ADD UNIQUE INDEX `file_version_application_unique` (`application_id`, `version`);
ALTER TABLE `application`
ADD COLUMN `disabled` BINARY(1) DEFAULT '0' NULL
AFTER `suggested_app`;
ALTER TABLE `user_content`
ADD COLUMN `bought` BINARY(1) DEFAULT '0' NULL
AFTER `bookmarked`;
ALTER TABLE `application`
ADD COLUMN `incomplete` BINARY(1) DEFAULT '1' NULL AFTER `disabled`;
ALTER TABLE `user`
CHANGE `name` `name` VARCHAR(45) CHARSET utf8 COLLATE utf8_persian_ci NULL,
CHANGE `family` `family` VARCHAR(45) CHARSET utf8 COLLATE utf8_persian_ci NULL;
ALTER TABLE `application`
CHANGE `name` `name` VARCHAR(255) CHARSET utf8 COLLATE utf8_persian_ci NULL;
ALTER TABLE `access`
CHANGE `label` `label` VARCHAR(50) CHARSET utf8 COLLATE utf8_persian_ci NULL;
ALTER TABLE `category`
CHANGE `name` `name` VARCHAR(255) CHARSET utf8 COLLATE utf8_persian_ci NULL;
ALTER TABLE `content`
CHANGE `title` `title` VARCHAR(255) CHARSET utf8 COLLATE utf8_persian_ci NULL;
ALTER TABLE `owner`
CHANGE `name` `name` VARCHAR(255) CHARSET utf8 COLLATE utf8_persian_ci NULL;
ALTER TABLE `role`
CHANGE `caption` `caption` VARCHAR(50) CHARSET utf8 COLLATE utf8_persian_ci NULL;
ALTER TABLE `user_group`
CHANGE `title` `title` VARCHAR(50) CHARSET utf8 COLLATE utf8_persian_ci NOT NULL;
ALTER TABLE `user`
CHANGE `address` `address` TEXT CHARSET utf8
COLLATE utf8_general_ci NULL;
ALTER TABLE `access_file`
CHANGE `access_id` `access_id` BIGINT(20) UNSIGNED NOT NULL;
ALTER TABLE `access_file`
ADD CONSTRAINT `access_file_access_to_access` FOREIGN KEY (`access_id`) REFERENCES `access` (`id`);
CREATE TABLE `deleted_user` (
`username` VARCHAR(50) CHARSET utf8 COLLATE utf8_bin NOT NULL,
`deletion_date` DATETIME NOT NULL,
`deleted_by` VARCHAR(50) CHARSET utf8 COLLATE utf8_bin NOT NULL,
`credit` INT(11),
`score` INT(11),
PRIMARY KEY (`username`)
);
INSERT INTO `user_group` (`id`, `title`, `logo_id`) VALUES ('1', 'عمومی', NULL);
ALTER TABLE `user_content`
ADD COLUMN `session` VARCHAR(100) NULL AFTER `bought`,
ADD UNIQUE INDEX `user_content_session_unique` (`session`);
ALTER TABLE `user_content`
DROP INDEX `user_content_session_unique`,
ADD UNIQUE INDEX `user_content_session_unique` (`session`, `content_id`);
CREATE TABLE `attempt` (
`session_id` VARCHAR(100) NOT NULL,
`time` DATETIME DEFAULT NULL,
`username` VARCHAR(50) DEFAULT NULL,
`captcha_passed` TINYINT(1) DEFAULT '0',
`remote_address` VARCHAR(30) DEFAULT NULL,
`device_info` VARCHAR(255) DEFAULT NULL,
`captcha_value` VARCHAR(30) DEFAULT NULL,
`true_password` TINYINT(1) DEFAULT '0',
PRIMARY KEY (`session_id`)
);
ALTER TABLE `attempt`
ADD COLUMN `count` INT(8) UNSIGNED DEFAULT 1
AFTER `true_password`;
ALTER TABLE `event`
ADD COLUMN `status` VARCHAR(3) NULL
AFTER `path`;
ALTER TABLE `event`
CHANGE `cookie` `cookie` VARCHAR(500) CHARSET utf8
COLLATE utf8_general_ci NULL;
ALTER TABLE `application`
ADD COLUMN `original_name` VARCHAR(128) CHARSET utf8
COLLATE utf8_general_ci NULL
AFTER `incomplete`;
ALTER TABLE `application`
ADD COLUMN `luncher` BINARY(1) DEFAULT '0' NULL
AFTER `original_name`,
ADD COLUMN `non_luncher` BINARY(1) DEFAULT '0' NULL
AFTER `luncher`;
ALTER TABLE `application`
DROP INDEX `unque`;
ALTER TABLE `application`
ADD INDEX `unique` (`app_package`, `luncher`);
ALTER TABLE `picture`
ADD COLUMN `luncher` BINARY(1) DEFAULT '0' NULL
AFTER `format`,
ADD COLUMN `non_luncher` BINARY(1) DEFAULT '0' NULL
AFTER `luncher`;
UPDATE picture p
SET luncher = (SELECT a.luncher
FROM `application` a
WHERE a.`banner_id` = p.`id`);
UPDATE picture p
SET non_luncher = (SELECT a.non_luncher
FROM `application` a
WHERE a.`banner_id` = p.`id`);
UPDATE picture p
SET published = FALSE
WHERE p.`content_id` IS NOT NULL AND (SELECT disabled
FROM application a
WHERE a.id = p.`content_id`) IS TRUE;
ALTER TABLE `application`
ADD COLUMN `status` INT(1) DEFAULT 1 NULL
AFTER `original_name`;
ALTER TABLE `picture`
ADD COLUMN `application_status` INT(1) DEFAULT 0 NULL
AFTER `format`;
ALTER TABLE `picture`
DROP COLUMN `luncher`,
DROP COLUMN `non_luncher`;
ALTER TABLE `application`
DROP COLUMN `luncher`,
DROP COLUMN `non_luncher`,
DROP INDEX `unique`;
ALTER TABLE `application`
ADD INDEX `unique` (`app_package`, `status`);
ALTER TABLE `picture`
CHANGE `application_status` `application_status` INT(1) DEFAULT 1 NULL;
CREATE TABLE `content_group` (
`id` BIGINT(20) UNSIGNED NOT NULL AUTO_INCREMENT,
`group_id` BIGINT(20) UNSIGNED NOT NULL,
`content_id` BIGINT(20) UNSIGNED NOT NULL,
PRIMARY KEY (`id`)
);
ALTER TABLE `content_group`
ADD CONSTRAINT `group_id_fk` FOREIGN KEY (`group_id`) REFERENCES `user_group` (`id`)
ON UPDATE CASCADE
ON DELETE CASCADE,
ADD CONSTRAINT `content_id_fk` FOREIGN KEY (`content_id`) REFERENCES `content` (`id`)
ON UPDATE CASCADE
ON DELETE CASCADE;
ALTER TABLE `content_group`
ADD UNIQUE INDEX `content_group_unique` (`group_id`, `content_id`);
CREATE TABLE `user_user_group` (
`id` BIGINT(20) UNSIGNED NOT NULL AUTO_INCREMENT,
`group_id` BIGINT(20) UNSIGNED NOT NULL,
`username` VARCHAR(50) NOT NULL,
PRIMARY KEY (`id`)
);
ALTER TABLE `user_user_group`
CHANGE `username` `username` VARCHAR(50) CHARSET utf8
COLLATE utf8_bin NOT NULL,
ADD CONSTRAINT `user_group_group_id_fk` FOREIGN KEY (`group_id`) REFERENCES `simorgh`.`user_group` (`id`)
ON UPDATE CASCADE
ON DELETE CASCADE,
ADD CONSTRAINT `user_group_username_fk` FOREIGN KEY (`username`) REFERENCES `simorgh`.`user` (`username`)
ON UPDATE CASCADE
ON DELETE CASCADE;
ALTER TABLE `user_user_group`
ADD UNIQUE INDEX `unique_relation` (`group_id`, `username`);
ALTER TABLE `user_group`
ADD COLUMN `default_group` BINARY(1) NULL
AFTER `logo_id`;
CREATE TABLE `spring_session` (
`PRIMARY_ID` char(36) DEFAULT NULL,
`SESSION_ID` char(36) NOT NULL,
`CREATION_TIME` bigint(20) NOT NULL,
`LAST_ACCESS_TIME` bigint(20) NOT NULL,
`MAX_INACTIVE_INTERVAL` int(11) NOT NULL,
`EXPIRY_TIME` bigint(20) DEFAULT NULL,
`PRINCIPAL_NAME` varchar(100) DEFAULT NULL,
PRIMARY KEY (`SESSION_ID`),
UNIQUE KEY `SPRING_SESSION_IX1` (`SESSION_ID`),
KEY `SPRING_SESSION_IX2` (`EXPIRY_TIME`),
KEY `SPRING_SESSION_IX3` (`PRINCIPAL_NAME`)
)
ENGINE = InnoDB
DEFAULT CHARSET = utf8
ROW_FORMAT = DYNAMIC;
CREATE TABLE `spring_session_attributes` (
`SESSION_ID` char(36) NOT NULL,
`ATTRIBUTE_NAME` varchar(200) NOT NULL,
`ATTRIBUTE_BYTES` blob NOT NULL,
PRIMARY KEY (`ATTRIBUTE_NAME`, `SESSION_ID`),
KEY `SPRING_SESSION_ATTRIBUTES_FK` (`SESSION_ID`),
CONSTRAINT `SPRING_SESSION_ATTRIBUTES_FK` FOREIGN KEY (`SESSION_ID`) REFERENCES `spring_session` (`SESSION_ID`)
ON DELETE CASCADE
)
ENGINE = InnoDB
DEFAULT CHARSET = utf8
ROW_FORMAT = DYNAMIC;
<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('HomeController', HomeController);
/** @ngInject */
function HomeController($log, homeService, principal) {
var vm = this;
vm.title = 'HomeController';
vm.dashboard = {};
activate();
////////////////
function activate() {
$log.debug('Home module activated');
principal.identity().then(function (data) {
vm.user = data;
vm.isAdmin = principal.hasRole('admin');
// console.log('home.isAdmin', vm.isAdmin);
getDashboard();
});
}
function getDashboard() {
if (vm.isAdmin) {
homeService.getDashboard()
.then(function (dashboard) {
vm.dashboard = dashboard;
});
} else {
homeService.getMyDashboard()
.then(function (dashboard) {
vm.dashboard = dashboard;
});
}
}
}
})();<file_sep>(function () {
'use strict';
angular
.module('app')
.factory('userService', userService);
/** @ngInject */
function userService(Upload, $http, host) {
var base = host + 'user/';
var service = {
getUsers: getUsers,
getAllUsers: getAllUser,
getUser: getUser,
getMe: getMe,
getAuthorities: getAuthorities,
createUser: createUser,
updateUser: updateUser,
deleteUser: deleteUser,
resetPassword: resetPassword,
updatePassword: updatePassword,
addCredit: addCredit,
saveProfilePicture: saveProfilePicture,
deleteProfilePicture: deleteProfilePicture,
getLastLogin: getLastLogin,
getAllSessions: getSessions,
getMySessions: getMySessions,
terminateSession: terminateSession,
usernameIsValid: usernameIsValid,
emailIsValid: emailIsValid,
mobilePhoneIsValid: mobilePhoneIsValid,
addGroup: addGroup,
removeGroup: removeGroup
};
return service;
function successCallback(response) {
return response.data;
}
////////////////
function createUser(user) {
return $http.post(base, user)
.then(successCallback);
}
function getUsers(params) {
return $http.get(base, {params: params})
.then(successCallback);
}
function getUser(username) {
var url = base + username;
return $http.get(url)
.then(successCallback);
}
function getAllUser() {
var url = base + 'all';
return $http.get(url)
.then(successCallback);
}
function getAuthorities() {
var url = base + 'authorities';
return $http.get(url)
.then(successCallback);
}
function updateUser(user) {
return $http.put(base, user)
.then(successCallback);
}
function resetPassword(username, password) {
var url = base + 'resetPassword?username=' + username;
return $http.put(url, password)
.then(successCallback);
}
function updatePassword(username, password, oldPassword) {
var url = base + 'updatePassword?username=' + username;
return $http.put(url, {password: <PASSWORD>, oldPassword: <PASSWORD>})
.then(successCallback);
}
function getMe() {
var url = base + 'me';
return $http.get(url)
.then(successCallback);
}
function addCredit(username, amount, comment) {
var url = base + username + '/addCredit?amount=' + amount;
if(comment)
url+='&comment='+comment;
return $http.put(url)
.then(successCallback);
}
function deleteUser(username) {
var url = base + username;
return $http.delete(url)
.then(successCallback);
}
function deleteProfilePicture(username) {
var url = base + username + '/picture';
return $http.delete(url)
.then(successCallback);
}
function getSessions(username, all) {
if (all === undefined) {
all = false;
}
var url = base + 'getAllSessions/' + username + '?all=' + all;
return $http.get(url)
.then(successCallback);
}
function getMySessions(all) {
if (all === undefined) {
all = false;
}
var url = base + 'getMySessions?all=' + all;
return $http.get(url)
.then(successCallback);
}
function getLastLogin(username) {
var url = base + 'getLastLogin/' + username;
return $http.get(url)
.then(successCallback);
}
function terminateSession(username, sessionId, all) {
if (all === undefined) {
all = false;
}
var url = base + 'deleteSessions/' + username + '?sessionId=' + sessionId + '&all=' + all;
return $http.delete(url)
.then(successCallback);
}
function saveProfilePicture(username, dataUrl) {
var url = base + username + '/picture';
return Upload.upload({
url: url,
file: Upload.dataUrltoBlob(dataUrl, 'image.png'),
method: 'POST'
})
.then(successCallback);
}
function usernameIsValid(username) {
return $http.get(base + 'usernameIsValid?username=' + username).then(successCallback);
}
function emailIsValid(email, username) {
var url = base + 'emailIsValid?email=' + email;
if (username)
url += '&username=' + username;
return $http.get(url).then(successCallback);
}
function mobilePhoneIsValid(mobilePhone, username) {
var url = base + 'mobilePhoneIsValid?mobilePhone=' + mobilePhone;
if (username)
url += '&username=' + username;
return $http.get(url).then(successCallback);
}
function addGroup(username, groupId) {
var url = base + username + '/addGroup';
return $http.put(url, {}, {params: {groupId: groupId}})
.then(successCallback);
}
function removeGroup(username, groupId) {
var url = base + username + '/removeGroup';
return $http.put(url, {}, {params: {groupId: groupId}})
.then(successCallback);
}
}
})();<file_sep>(function () {
'use strict';
/**
* @ngdoc directive
* @name app.directive:samatHeader
* @description
* # samatHeader
*/
angular
.module('app')
.directive('navbar', samatHeader);
/** @ngInject */
function samatHeader() {
return {
templateUrl: 'app/main/navbar/navbar.html',
restrict: 'E',
replace: true,
controller: samatHeaderController,
controllerAs: 'nav'
};
function samatHeaderController($uibModal, host, principal, $state, $stateParams) {
var nav = this;
nav.openRegisterForm = openRegisterForm;
nav.signOut = signOut;
nav.downloadSimorgh = host + 'download/simorgh/ir.simorghmarket.simorgh.apk';
nav.humClick = function () {
if ($('#bs-example-navbar-collapse-1').hasClass('collapse'))
$('#bs-example-navbar-collapse-1').removeClass('collapse', 5000, 'swing');
else
$('#bs-example-navbar-collapse-1').addClass('collapse', 1000, 'swing');
};
console.log('nav activated');
/*principal.identity().then(function () {
nav.user = principal.getIdentity();
});*/
function signOut() {
principal.logout().then(function () {
$state.go($state.current.name, $stateParams, {reload: true});
})
}
function openRegisterForm() {
var modal = $uibModal.open(
{
animation: true,
templateUrl: 'app/components/home/register/register.html',
controller: 'RegisterController',
controllerAs: 'reg',
size: 'md'
// appendTo: parentElem,
}
);
}
}
}
})();
<file_sep>package ir.saafta.model;
import javax.persistence.*;
/**
* Created by reza on 11/14/16.
*/
public class AccessFile {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@ManyToOne
@JoinColumn(name = "access_id")
private Access access;
/*@ManyToOne
@JoinColumn(name = "file_id")
private File file;*/
private Long fileId;
public AccessFile(Access access, Long fileId) {
this.access = access;
this.fileId = fileId;
}
public AccessFile() {
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public Access getAccess() {
return access;
}
public void setAccess(Access access) {
this.access = access;
}
/* public File getFile() {
return file;
}
public void setFile(File file) {
this.file = file;
}*/
public Long getFileId() {
return fileId;
}
public void setFileId(Long fileId) {
fileId = fileId;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof AccessFile)) return false;
AccessFile that = (AccessFile) o;
return getFileId().equals(that.getFileId()) && getAccess().equals(that.getAccess());
}
}
<file_sep>(function () {
'use strict';
angular
.module('app', [
//third party
'angular-loading-bar',
'angular-confirm',
'ADM-dateTimePicker',
'ngAnimate',
'ngSanitize',
'ngTable',
'pascalprecht.translate',
'toastr',
'ui.bootstrap',
'ui.router',
'ui.select',
'ngFileUpload',
'ngImgCrop',
'angular-img-cropper',
'slickCarousel',
'ngMaterial',
//our modules
'auth'
]);
})();
<file_sep>package ir.saafta.web;
import ir.saafta.model.Access;
import ir.saafta.repo.AccessRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.security.access.prepost.PreAuthorize;
import org.springframework.web.bind.annotation.*;
/**
* Created by reza on 11/14/16.
*/
@RestController
@RequestMapping("/access")
public class AccessController {
private final AccessRepository accessRepository;
@Autowired
public AccessController(AccessRepository accessRepository) {
this.accessRepository = accessRepository;
}
@RequestMapping(method = RequestMethod.GET)
public Page<Access> get(Pageable pageable) {
return accessRepository.findAll(pageable);
}
@RequestMapping(value = "/{id}", method = RequestMethod.GET)
public Access getById(@PathVariable Long id) {
return accessRepository.findOne(id);
}
@RequestMapping(value = "/all", method = RequestMethod.GET)
public Iterable<Access> getAll() {
return accessRepository.findAll();
}
@PreAuthorize("hasAuthority('admin')")
@RequestMapping(method = RequestMethod.POST)
public Access create(@RequestBody Access access) {
access.setId(null);
return accessRepository.save(access);
}
@PreAuthorize("hasAuthority('admin')")
@RequestMapping(method = RequestMethod.PUT)
public Access update(@RequestBody Access access) {
return accessRepository.save(access);
}
@PreAuthorize("hasAuthority('admin')")
@RequestMapping(value = "/{id}", method = RequestMethod.DELETE)
public void delete(@PathVariable Long id) {
try {
accessRepository.delete(id);
} catch (org.springframework.dao.DataIntegrityViolationException e) {
throw new RuntimeException("Access can not be deleted due to depended information");
}
}
}
<file_sep>package ir.saafta.model;
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class SpringSession {
@Id
private String sessionId;
private String principalName;
public String getSessionId() {
return sessionId;
}
public void setSessionId(String sessionId) {
this.sessionId = sessionId;
}
public String getPrincipalName() {
return principalName;
}
public void setPrincipalName(String principalName) {
this.principalName = principalName;
}
}
<file_sep>(function () {
'use strict';
angular
.module('app')
.factory('userGroupService', userGroupService);
/** @ngInject */
function userGroupService($log, $http, host) {
var base = host + 'userGroup/';
var service = {
getUserGroups: getUserGroups,
getAllUserGroups: getAllUserGroups,
getUserGroup: getUserGroup,
createUserGroup: createUserGroup,
updateUserGroup: updateUserGroup,
deleteUserGroup: deleteUserGroup,
addUserToGroup: addUserToGroup,
removeUserFromGroup: removeUserFromGroup,
getNonGroupUsers: getNonGroupUsers
};
return service;
function successCallback(response) {
return response.data;
}
////////////////
function createUserGroup(userGroup) {
return $http.post(base, userGroup)
.then(successCallback);
}
function getUserGroups(params) {
return $http.get(base, {params: params})
.then(successCallback);
}
function getAllUserGroups() {
var url = base + 'all';
return $http.get(url)
.then(successCallback);
}
function getUserGroup(id) {
var url = base + id;
return $http.get(url)
.then(successCallback);
}
function updateUserGroup(userGroup) {
return $http.put(base, userGroup)
.then(successCallback);
}
function deleteUserGroup(id) {
var url = base + id;
return $http.delete(url)
.then(successCallback);
}
function addUserToGroup(groupId, username) {
var url = base + groupId + '/addUser/' + username;
return $http.put(url)
.then(successCallback);
}
function removeUserFromGroup(groupId, username) {
var url = base + groupId + '/removeUser/' + username;
return $http.delete(url)
.then(successCallback);
}
function getGroupUsers(groupId) {
var url = base + groupId + '/getUsers';
return $http.get(url)
.then(successCallback);
}
function getNonGroupUsers(groupId) {
var url = base + groupId + '/getNonUsers';
return $http.get(url)
.then(successCallback);
}
}
})();<file_sep>/*
SQLyog Ultimate v11.11 (64 bit)
MySQL - 5.7.10-log : Database - sbazaar
*********************************************************************
*/
/*!40101 SET NAMES utf8 */;
/*!40101 SET SQL_MODE=''*/;
/*!40014 SET @OLD_UNIQUE_CHECKS=@@UNIQUE_CHECKS, UNIQUE_CHECKS=0 */;
/*!40014 SET @OLD_FOREIGN_KEY_CHECKS=@@FOREIGN_KEY_CHECKS, FOREIGN_KEY_CHECKS=0 */;
/*!40101 SET @OLD_SQL_MODE=@@SQL_MODE, SQL_MODE='NO_AUTO_VALUE_ON_ZERO' */;
/*!40111 SET @OLD_SQL_NOTES=@@SQL_NOTES, SQL_NOTES=0 */;
CREATE DATABASE /*!32312 IF NOT EXISTS*/`sbazaar` /*!40100 DEFAULT CHARACTER SET utf8 */;
USE `sbazaar`;
/*Data for the table `authorities` */
insert into `authorities`(`username`,`authority`) values ('ali','user'),('asghar','user'),('reza','admin'),('reza','supervisior'),('saeid','supervisior');
/*Data for the table `role` */
insert into `role`(`authority`,`caption`) values ('admin','مدیر'),('supervisior','ناظر'),('user','کاربر');
/*Data for the table `user` */
insert into `user`(`username`,`password`,`enabled`,`name`,`family`,`address`,`mobile_phone`,`mobile_phone_two`,`mobile_phone_three`,`phone`,`postal_code`,`email`,`profile_id`,`credit`,`score`,`imei`) values ('ali','$2a$10$0IGNngkmz19I1Gn68BJwheCQuj1qrWevXM8v7hqWQl6bgsXTrdX2q',1,'علی','دایی','تهران قیطریه','09121156384',NULL,NULL,'02122336598','1548632475','<EMAIL>',NULL,39000,0,NULL),('asghar','$2a$10$lFNmWmTGovkVDar4OkPRH.AqHeQYCtrAN3dgaBEpYD0ouXzbYa2ay',1,'اصغر','چامسکی',NULL,'09123457895',NULL,NULL,NULL,NULL,'<EMAIL>',NULL,NULL,NULL,NULL),('reza','$2a$10$dK2RFmH.JYBzjChtKF6iberczBmCfchHadKw0FDHtOIB4kcT7HT0a',1,'رضا','کشمیر','کرج هفت تیر','09126542136',NULL,NULL,NULL,NULL,'<EMAIL>',NULL,0,0,NULL),('saeid','$2a$10$LaNTXEzjB8EF3guAohmzaOZ08sV4G/EsvVcy1Hb1vHGnNWEgOxwEG',1,'سعید','جاجیزاده',NULL,'0912765438910',NULL,NULL,NULL,NULL,'<EMAIL>',NULL,0,0,NULL);
/*!40101 SET SQL_MODE=@OLD_SQL_MODE */;
/*!40014 SET FOREIGN_KEY_CHECKS=@OLD_FOREIGN_KEY_CHECKS */;
/*!40014 SET UNIQUE_CHECKS=@OLD_UNIQUE_CHECKS */;
/*!40111 SET SQL_NOTES=@OLD_SQL_NOTES */;
<file_sep>(function () {
'use strict';
/**
* @ngdoc directive
* @name app.directive:samatHeader
* @description
* # samatHeader
*/
angular
.module('app')
.directive('saaftaHeader', samatHeader);
/** @ngInject */
function samatHeader() {
return {
templateUrl: 'app/main/header/header.html',
restrict: 'E',
replace: true,
controllerAs: 'vm',
controller: HeaderController
};
/** @ngInject */
function HeaderController(homeService, host, $state, $timeout, $mdMedia, $scope) {
/* jshint validthis: true */
var vm = this;
vm.myInterval = 5000;
vm.noWrapSlides = false;
vm.active = 1;
vm.host = host;
vm.slides = [];
vm.show = $state.current.name == "root.home";
vm.screenIsGreaterThanSmall = $mdMedia('gt-sm');
vm.screenIsSmall = !vm.screenIsGreaterThanSmall;
vm.boxHeight = vm.screenIsGreaterThanSmall ? {'height': '320px'} : {'height': '190px'};
homeService.getPublishedBanners().then(function (data) {
vm.slides = data;
var width = (window.innerWidth + 6) / 3;
vm.slides.forEach(function (slide) {
slide.styles = {
"width": width + "px",
"background": "rgba(0,0,0,0) url('" + host + slide.url + "') no-repeat center center",
"background-size": "cover",
"-webkit-background-size": "cover",
"-moz-background-size": "cover",
"-o-background-size": "cover"
}
});
$timeout(function () {
makeSlider();
}, 1000);
});
$scope.$watch(function () {
return $mdMedia('gt-sm');
}, function (gt_sm) {
console.debug('Watch small:', gt_sm, window.innerWidth);
vm.screenIsSmall = !gt_sm;
vm.screenIsGreaterThanSmall = gt_sm;
vm.boxHeight = vm.screenIsGreaterThanSmall ? {'height': '320px'} : {'height': '190px'};
if (vm.carousel) {
vm.carouselAPI.destroy();
makeSlider();
}
if (vm.screenIsGreaterThanSmall)
console.debug('It is greater than small!', window.innerWidth);
else if (vm.screenIsSmall)
console.debug('It is small!', window.innerWidth);
});
function coverflow(data) {
data.current.removeClass('pre following')
.nextAll()
.removeClass('pre following')
.addClass('following')
.end()
.prevAll()
.removeClass('pre following')
.addClass('pre');
}
function makeSlider() {
/*$('.owl-carousel').owlCarousel({
margin: 2,
loop: true,
autoWidth: true,
items: 3,
rtl: true,
autoplay: true,
autoplayTimeout: 3000
});*/
vm.carousel = $('#Carousel').glide({
type: 'carousel',
paddings: vm.screenIsSmall ? '0px' : '25%',
startAt: 2,
afterInit: function (data) {
coverflow(data);
},
afterTransition: function (data) {
coverflow(data);
}
});
vm.carouselAPI = vm.carousel.data('glide_api');
}
}
}
})();
<file_sep>(function () {
'use strict';
angular
.module('app')
.factory('applicationService', applicationService);
/** @ngInject */
function applicationService($log, $http, host, Upload) {
var base = host + 'application/';
var service = {
getApplications: getApplications,
getSimurgh: getSimurgh,
getApplication: getApplication,
getFiles: getFiles,
deleteFile: deleteFile,
deleteBanner: deleteBanner,
createApplication: createApplication,
updateApplication: updateApplication,
updateLogo: updateLogo,
updateBanner: updateBanner,
addPic: addPic,
deletePic: deletePic,
deleteApplication: deleteApplication,
getActiveVersion: getActiveVersion,
setActiveVersion: setActiveVersion,
addGroup: addGroup,
removeGroup: removeGroup,
mostSeen: mostSeen
};
return service;
function successCallback(response) {
return response.data;
}
////////////////
function createApplication(application) {
return $http.post(base, application)
.then(successCallback);
}
function getFiles(id) {
var url = base + id + '/files';
return $http.get(url)
.then(successCallback);
}
function mostSeen() {
var url = base + 'mostSeen';
return $http.get(url)
.then(successCallback);
}
function deleteFile(id, fileId) {
var url = base + id + '/file/' + fileId;
return $http.delete(url)
.then(successCallback);
}
function deleteBanner(id, fileId) {
var url = base + id + '/banner/';
return $http.delete(url)
.then(successCallback);
}
function getActiveVersion(id) {
var url = base + id + '/getActiveVersion';
return $http.get(url)
.then(successCallback);
}
function setActiveVersion(id, fileId) {
var url = base + id + '/setActiveVersion';
var params = {
fileId: fileId
};
return $http.put(url, {}, {params: params})
.then(successCallback);
}
function getApplications(data, params) {
params.manage = true;
var p = Object.keys(params).map(function (k) {
return encodeURIComponent(k) + '=' + encodeURIComponent(params[k])
}).join('&');
console.log(p);
return $http.put(base + '?' + p, data)
.then(successCallback);
}
function getSimurgh(type) {
var url = base + 'simorgh';
return $http.get(url, {params: {type: type}})
.then(successCallback);
}
function getApplication(id) {
var url = base + id + '?manage=true';
return $http.get(url)
.then(successCallback);
}
function updateApplication(application) {
return $http.put(base + application.id, application)
.then(successCallback);
}
function updateLogo(id, dataUrl) {
var url = base + id + '/logo';
return Upload.upload({
url: url,
file: Upload.dataUrltoBlob(dataUrl, 'image.png'),
method: 'POST'
})
.then(successCallback);
}
function updateBanner(id, dataUrl) {
var url = base + id + '/banner';
return Upload.upload({
url: url,
file: Upload.dataUrltoBlob(dataUrl, 'image.jpg'),
method: 'POST'
})
.then(successCallback);
}
function addPic(id, dataUrl, label) {
var url = base + id + '/picture';
return Upload.upload({
url: url,
data: {file: Upload.dataUrltoBlob(dataUrl, 'image.jpg'), label: label},
method: 'POST'
})
.then(successCallback);
}
function deletePic(id, picId) {
var url = base + id + "/picture/" + picId;
return $http.delete(url)
.then(successCallback);
}
function deleteApplication(id) {
var url = base + id;
return $http.delete(url)
.then(successCallback);
}
function addGroup(id, groupId) {
var url = base + id + '/addGroup';
var params = {
groupId: groupId
};
return $http.put(url, {}, {params: params})
.then(successCallback);
}
function removeGroup(id, groupId) {
var url = base + id + "/removeGroup/";
return $http.delete(url, {params: {groupId: groupId}})
.then(successCallback);
}
}
})();<file_sep>(function () {
'use strict';
/**
* @ngdoc directive
* @name app.directive:headerNotification
* @description
* # headerNotification
*/
angular
.module('app')
.directive('headerNotification', headerNotification);
/** @ngInject */
function headerNotification() {
return {
bindToController: true,
controller: headerCtrl,
controllerAs: 'vm',
templateUrl: 'app/main/header/header-notification/header-notification.html',
restrict: 'E',
replace: true,
scope: {}
};
}
/** @ngInject */
function headerCtrl($translate, host, principal, $rootScope) {
var vm = this;
vm.host = host;
vm.Languages = [
{
key: 'fa_IR',
title: 'APP.LANG.FA_IR'
},
{
key: 'en_US',
title: 'APP.LANG.EN_US'
}
];
vm.logout = logout;
vm.toggleNav = toggleNav;
principal.identity().then(function (data) {
// console.log('header notification, identity', data);
vm.user = data;
});
vm.changeLanguage = changeLanguage;
function changeLanguage(langKey) {
$translate.use(langKey);
}
function logout() {
principal.logout();
}
function toggleNav() {
console.log('toggleNav', $rootScope.showNav);
$rootScope.showNav = !$rootScope.showNav;
}
}
})();<file_sep>(function () {
'use strict';
angular
.module('app')
.filter('eNumber', eNumber)
.filter('deviceType', deviceType)
.filter('fileLogAction', fileLogAction)
.filter('incomeDate', incomeDate)
.filter('jDate', jDate)
.filter('jDateAndTime', jDateAndTime)
.filter('pCurrency', pCurrency)
.filter('pNumber', pNumber)
.filter('sDate', sDate)
.filter('trueFalse', trueFalse)
.filter('trustedHtml', trustedHtml)
.filter('trustedUrl', trustedUrl)
.filter('sessionStatus', sessionStatus)
;
/** @ngInject */
function eNumber() {
return eNumberFilter;
////////////////
function eNumberFilter(value) {
if (angular.isDefined(value) && value !== null && value.toString().trim() !== '') {
var persianNumbers = ['۱', '۲', '۳', '۴', '۵', '۶', '۷', '۸', '۹', '۰'];
var englishNumbers = ['1', '2', '3', '4', '5', '6', '7', '8', '9', '0'];
for (var i = 0, numbersLen = persianNumbers.length; i < numbersLen; i += 1) {
value = value.toString().replace(new RegExp(persianNumbers[i], 'g'), englishNumbers[i]);
}
}
return value;
}
}
function deviceType() {
return deviceTypeFilter;
////////////////
function deviceTypeFilter(type) {
return type === 1 ? 'ATM' : 'UPS';
}
}
function fileLogAction() {
return fileLogActionFilter;
////////////////
function fileLogActionFilter(type) {
return type === 1 ? 'APP.ACTIONS.DELETE' : 'APP.ACTIONS.UPLOAD';
}
}
/** @ngInject */
function incomeDate(moment) {
return incomeDateFilter;
////////////////
function incomeDateFilter(date) {
if (angular.isUndefined(date) || date === null) {
return 'نامشخص ';
}
return moment(date).format('jMMMM jYY').replace('امرداد', 'مرداد');
}
}
/** @ngInject */
function jDate(moment) {
return jDateFilter;
////////////////
function jDateFilter(date) {
if (angular.isUndefined(date) || date === null) {
return 'نامشخص ';
}
return moment(date).format('jD jMMMM jYY ').replace('امرداد', 'مرداد');
}
}
/** @ngInject */
function jDateAndTime(moment) {
return jDateAndTimeFilter;
////////////////
function jDateAndTimeFilter(date) {
if (angular.isUndefined(date) || date === null) {
return 'نامشخص ';
}
return moment(date).format('jD jMMMM jYY - hh:mm:ss').replace('امرداد', 'مرداد');
}
}
function pCurrency() {
return pCurrencyFilter;
////////////////
function pCurrencyFilter(number, giveZero) {
if (number === null || angular.isUndefined(number)) {
return '';
}
if (number === 0) {
if (giveZero) {
return '۰';
}
return 'رایگان';
}
var parts = number.toString().split('.');
parts[0] = parts[0].replace(/\B(?=(\d{3})+(?!\d))/g, ',');
return pNumber()(parts.join('.')) + ' تومان';
}
}
function pNumber() {
return pNumberFilter;
////////////////
function pNumberFilter(value) {
if (angular.isDefined(value) && value !== null && value.toString().trim() !== '') {
var persianNumbers = ['۱', '۲', '۳', '۴', '۵', '۶', '۷', '۸', '۹', '۰'];
var englishNumbers = ['1', '2', '3', '4', '5', '6', '7', '8', '9', '0'];
for (var i = 0, numbersLen = englishNumbers.length; i < numbersLen; i += 1) {
value = value.toString().replace(new RegExp(englishNumbers[i], 'g'), persianNumbers[i]);
}
return value;
}
else {
return '';
}
}
}
/** @ngInject */
function sDate(moment) {
return sDateFilter;
////////////////
function sDateFilter(date) {
return date ? moment(date, 'jYYYY-jMM-jDD').format('YYYY-MM-DD') : null;
}
}
function trueFalse() {
return trueFalseFilter;
////////////////
function trueFalseFilter(flag) {
return flag ? 'APP.SHARE.TRUE' : 'APP.SHARE.FALSE';
}
}
/** @ngInject */
function trustedHtml($sce) {
return trustedHtmlFilter;
////////////////
function trustedHtmlFilter(url) {
return $sce.trustAsHtml(url);
}
}
/** @ngInject */
function trustedUrl($sce) {
return trustedUrlFilter;
////////////////
function trustedUrlFilter(url) {
return $sce.trustAsResourceUrl(url);
}
}
/** @ngInject */
function sessionStatus() {
var statuses = {
'ACTIVE': 'فعال',
'SIGNED_OUT': 'خارج شده',
'EXPIRED': 'منقضی شده',
'TERMINATED': 'متوقف شده'
};
return sessionStatusFilter;
////////////////
function sessionStatusFilter(session) {
return statuses[session.status];
}
}
})();
<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('BannerController', BannerController);
/** @ngInject */
function BannerController($log, $scope, $ngConfirm, NgTableParams, bannerService, host, util) {
var vm = this;
vm.title = 'BannerController';
vm.host = host;
vm.bannerTable = new NgTableParams({
page: 1,
count: 20
}, {
getData: getBanners
});
vm.delete = Delete;
vm.publish = publish;
vm.update = update;
activate();
////////////////
function activate() {
$log.debug('Banner module activated');
}
function Delete(id) {
$ngConfirm({
title: 'حذف بنر',
content: '<span class="text-danger">{{"Are you sure about delete this item?"|translate}}</span>',
scope: $scope,
buttons: {
sayBoo: {
text: 'بله',
btnClass: 'btn-blue',
action: function (scope, button) {
vm.loading = true;
bannerService.deleteBanner(id)
.then(function () {
util.toast('success', 'بنر با موفقیت حذف شد.');
vm.loading = false;
vm.bannerTable.reload();
}, function () {
util.toast('error', 'خطا در حذف بنر!');
vm.loading = false;
});
return true;
}
},
somethingElse: {
text: 'خیر',
btnClass: 'btn-orange',
action: function (scope, button) {
return true;
}
}
}
});
}
function getBanners(tabelparams) {
var params = {
page: tabelparams.page() - 1,
size: tabelparams.count()
};
vm.loading = true;
return bannerService.getBanners(params).then(function (data) {
vm.loading = false;
tabelparams.total(data.totalElements);
return data.content;
});
}
function publish(banner) {
vm.loading = true;
bannerService.publishBanner(banner.id, !banner.published)
.then(function (response) {
vm.loading = false;
banner.published = response.data.published;
util.toast('success', 'تغییر وضعیت نمایش بنر');
}, function (error) {
vm.loading = false;
util.toast('error', error.data.message);
});
}
function update(banner) {
vm.loading = true;
bannerService.updateBanner(banner.id, banner.label)
.then(function () {
vm.loading = false;
banner.editing = false;
util.toast('success', 'عنوان بنر با موفقیت ویرایش شد.');
}, function () {
vm.loading = false;
vm.bannerTable.reload();
util.toast('error', 'خطا در ویرایش عنوان بنر!');
});
}
}
})();<file_sep>package ir.saafta.model;
import javax.persistence.*;
import java.util.Date;
/**
* Created by Reza on 4/2/2017.
*/
@Entity
@Inheritance(strategy = InheritanceType.JOINED)
public class UserContent {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@ManyToOne
@JoinColumn(name = "username")
private User user;
@ManyToOne
@JoinColumn(name = "contentId")
private Content content;
private String phoneNumber;
private String session;
private Double rating;
private Date installDate;
private Date uninstallDate;
private String currentVersion;
private Boolean bookmarked;
private Boolean bought;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public User getUser() {
return user;
}
public void setUser(User user) {
this.user = user;
}
public Content getContent() {
return content;
}
public void setContent(Content content) {
this.content = content;
}
public String getPhoneNumber() {
return phoneNumber;
}
public void setPhoneNumber(String phoneNumber) {
this.phoneNumber = phoneNumber;
}
public Double getRating() {
return rating;
}
public void setRating(Double rating) {
this.rating = rating;
}
public Date getInstallDate() {
return installDate;
}
public void setInstallDate(Date installDate) {
this.installDate = installDate;
}
public Date getUninstallDate() {
return uninstallDate;
}
public void setUninstallDate(Date uninstallDate) {
this.uninstallDate = uninstallDate;
}
public String getCurrentVersion() {
return currentVersion;
}
public void setCurrentVersion(String currentVersion) {
this.currentVersion = currentVersion;
}
public Boolean getBookmarked() {
return bookmarked;
}
public void setBookmarked(Boolean bookmarked) {
this.bookmarked = bookmarked;
}
public Boolean getBought() {
return bought;
}
public void setBought(Boolean bought) {
this.bought = bought;
}
public String getSession() {
return session;
}
public void setSession(String session) {
this.session = session;
}
}
<file_sep>package ir.saafta.repo;
import com.sun.jersey.core.impl.provider.entity.XMLRootObjectProvider;
import ir.saafta.dto.ApplicationStatus;
import ir.saafta.model.*;
import org.springframework.data.jpa.domain.Specification;
import javax.persistence.Id;
import javax.persistence.criteria.*;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Date;
import java.util.List;
/**
* Created by reza on 12/19/16.
*/
public class LightApplicationSpec implements Specification<LightApplication> {
private String simurghPackage;
private Category category;
private Long ownerId;
private String appPackage;
private Integer fee;
private String name;
private Date createDate;
private Date lastUpdate;
private Boolean specificApp;
private String username;
private Boolean suggestedApp;
private Boolean newApp;
private List<ApplicationStatus> statuses;
private UserGroup group;
private List<UserGroup> groups;
public LightApplicationSpec(String simurghPackage, Category category, Long ownerId, String appPackage, Integer fee,
String name, Date createDate, Date lastUpdate, Boolean specificApp, String username,
List<ApplicationStatus> statuses) {
this.category = category;
this.ownerId = ownerId;
this.simurghPackage = simurghPackage;
this.appPackage = appPackage;
this.fee = fee;
this.name = name;
this.createDate = createDate;
this.lastUpdate = lastUpdate;
this.specificApp = specificApp;
this.username = username;
this.statuses = statuses;
}
public LightApplicationSpec() {
}
public LightApplicationSpec(String simurghPackage, Category category, Long ownerId, String appPackage, Integer fee,
String name, Date createDate, Date lastUpdate, Boolean specificApp, String username,
Boolean suggestedApp, Boolean newApp, List<ApplicationStatus> statuses) {
this.category = category;
this.ownerId = ownerId;
this.simurghPackage = simurghPackage;
this.appPackage = appPackage;
this.fee = fee;
this.name = name;
this.createDate = createDate;
this.lastUpdate = lastUpdate;
this.specificApp = specificApp;
this.username = username;
this.suggestedApp = suggestedApp;
this.newApp = newApp;
this.statuses = statuses;
}
@Override
public Predicate toPredicate(Root<LightApplication> root, CriteriaQuery<?> query, CriteriaBuilder cb) {
Predicate result = cb.notEqual(root.get("appPackage"), simurghPackage);
if (category != null) {
result = cb.and(result, cb.equal(root.get("category"), category));
}
if (ownerId != null) {
result = cb.and(result, cb.equal(root.get("ownerId"), ownerId));
}
if (username != null) {
result = cb.and(result, cb.equal(root.get("userId"), username));
}
if (appPackage != null) {
result = cb.and(result, cb.like(root.get("appPackage"), "%" + appPackage + "%"));
}
if (fee != null) {
result = cb.and(result, cb.equal(root.get("fee"), fee));
}
if (name != null) {
result = cb.and(result, cb.like(root.get("name"), "%" + name + "%"));
}
if (createDate != null) {
result = cb.and(result, cb.equal(root.get("createDate"), createDate));
}
if (lastUpdate != null) {
result = cb.and(result, cb.equal(root.get("lastUpdate"), lastUpdate));
}
if (specificApp != null) {
result = cb.and(result, cb.equal(root.get("specificApp"), specificApp));
}
if (suggestedApp != null) {
result = cb.and(result, cb.equal(root.get("suggestedApp"), suggestedApp));
}
if (newApp != null) {
result = cb.and(result, cb.equal(root.get("newApp"), newApp));
}
//noinspection Duplicates
if (statuses != null && statuses.size() > 0) {
result = cb.and(result, root.get("status").in(statuses));
}
if (group != null) {
// see if requested group is one of the application's groups
Subquery<ContentGroup> subQuery2 = query.subquery(ContentGroup.class); // sub-query instance with ContentGroup type
Root<ContentGroup> rootContentGroup2 = subQuery2.from(ContentGroup.class); // subRoot instance with ContentGroup type
Predicate isEqual2 = cb.equal(rootContentGroup2.get("contentId"), root.get("id"));
Predicate isEqual3 = cb.equal(rootContentGroup2.get("groupId"), group.getId());
subQuery2.select(rootContentGroup2).where(isEqual2, isEqual3); // select all ContentGroup correlated with the application and the group
Predicate isMember = cb.exists(subQuery2); // see if application is in the same group
result = cb.and(result, isMember);
}
// if we should care for application group
if (groups != null) {
// see if application has any group
Subquery<ContentGroup> subQuery = query.subquery(ContentGroup.class); // sub-query instance with ContentGroup type
Root<ContentGroup> rootContentGroup = subQuery.from(ContentGroup.class); // subRoot instance with ContentGroup type
Predicate isEqual = cb.equal(rootContentGroup.get("contentId"), root.get("id")); // sub-query condition: all ContentGroup correlated with the application
subQuery.select(rootContentGroup).where(isEqual); // select all ContentGroup correlated with the application
Predicate isEmpty = cb.not(cb.exists(subQuery)); // see if application has any group
if (!groups.isEmpty()) {
// see if requested group is one of the application's groups
Subquery<ContentGroup> subQuery2 = query.subquery(ContentGroup.class); // sub-query instance with ContentGroup type
Root<ContentGroup> rootContentGroup2 = subQuery2.from(ContentGroup.class); // subRoot instance with ContentGroup type
Predicate isEqual2 = cb.equal(rootContentGroup2.get("contentId"), root.get("id"));
List<Long> Ids = new ArrayList<>();
for (UserGroup g : groups) {
Ids.add(g.getId());
}
Predicate isIn = rootContentGroup2.get("groupId").in(Ids);
subQuery2.select(rootContentGroup2).where(isEqual2, isIn); // select all ContentGroup correlated with the application and the group
Predicate isMember = cb.exists(subQuery2); // see if application is in the same group
// so we want all applications which has either the first condition `isEmpty` or the second one `isMember`
Predicate orClause = cb.or(isEmpty, isMember);
// the OR clause would be one of the final result conditions
result = cb.and(result, orClause);
} else {
result = cb.and(result, isEmpty);
}
}
return result;
}
public String getSimurghPackage() {
return simurghPackage;
}
public void setSimurghPackage(String simurghPackage) {
this.simurghPackage = simurghPackage;
}
public Category getCategory() {
return category;
}
public void setCategory(Category category) {
this.category = category;
}
public Long getOwnerId() {
return ownerId;
}
public void setOwnerId(Long ownerId) {
this.ownerId = ownerId;
}
public String getAppPackage() {
return appPackage;
}
public void setAppPackage(String appPackage) {
this.appPackage = appPackage;
}
public Integer getFee() {
return fee;
}
public void setFee(Integer fee) {
this.fee = fee;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Date getCreateDate() {
return createDate;
}
public void setCreateDate(Date createDate) {
this.createDate = createDate;
}
public Date getLastUpdate() {
return lastUpdate;
}
public void setLastUpdate(Date lastUpdate) {
this.lastUpdate = lastUpdate;
}
public Boolean getSpecificApp() {
return specificApp;
}
public void setSpecificApp(Boolean specificApp) {
this.specificApp = specificApp;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public Boolean getSuggestedApp() {
return suggestedApp;
}
public void setSuggestedApp(Boolean suggestedApp) {
this.suggestedApp = suggestedApp;
}
public Boolean getNewApp() {
return newApp;
}
public void setNewApp(Boolean newApp) {
this.newApp = newApp;
}
public List<ApplicationStatus> getStatuses() {
return statuses;
}
public void setStatuses(List<ApplicationStatus> statuses) {
this.statuses = statuses;
}
public UserGroup getGroup() {
return group;
}
public void setGroup(UserGroup group) {
this.group = group;
}
public List<UserGroup> getGroups() {
return groups;
}
public void setGroups(List<UserGroup> groups) {
this.groups = groups;
}
}
<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('HomeController', HomeController);
/** @ngInject */
function HomeController($log, homeService, host, applicationService) {
var vm = this;
vm.host = host;
vm.title = 'HomeController';
activate();
////////////////
function activate() {
$log.debug('Home module activated');
getBanners();
getSpecifics();
}
this.carouselOne = [
{
"image": "assets/images/carousels/slide-1.jpg",
"title": "Carousel Caption One"
}, {
"image": "assets/images/carousels/slide-2.jpg",
"title": "Carousel Caption Two"
}, {
"image": "assets/images/carousels/slide-3.jpg",
"title": "Carousel Caption Three"
}
];
this.carouselTwo = [
'assets/images/carousels/slide-4.jpg',
'assets/images/carousels/slide-5.jpg',
'assets/images/carousels/slide-6.jpg'
];
this.carouselThree = [
'assets/images/carousels/slide-sm-1.jpg',
'assets/images/carousels/slide-sm-2.jpg',
'assets/images/carousels/slide-sm-3.jpg',
'assets/images/carousels/slide-sm-4.jpg',
'assets/images/carousels/slide-sm-5.jpg',
'assets/images/carousels/slide-sm-6.jpg',
'assets/images/carousels/slide-sm-7.jpg',
'assets/images/carousels/slide-sm-8.jpg'
];
function getBanners() {
homeService.getPublishedBanners().then(function (data) {
vm.banners = data;
})
}
function getSpecifics() {
applicationService.getSpecificApplications().then(function (data) {
vm.specifics = data;
})
}
}
})();<file_sep>(function () {
'use strict';
angular
.module('app')
.factory('newsService', newsService);
/** @ngInject */
function newsService($log, $http, host, Upload) {
var base = host + 'news/';
var service = {
getNewsList: getNewsList,
getAllNews: getAllNews,
getCurrentNews: getCurrentNews,
getNews: getNews,
createNews: createNews,
updateNews: updateNews,
deleteNews: deleteNews,
publishNews: publishNews,
adClicked: adClicked
};
return service;
function successCallback(response) {
return response.data;
}
////////////////
function createNews(data, dataUrl) {
data.file = Upload.dataUrltoBlob(dataUrl, 'image.jpg');
return Upload.upload({
url: base,
data: data,
method: 'POST'
});
}
function getNewsList(params) {
return $http.get(base, {params: params})
.then(successCallback);
}
function getAllNews() {
var url = base + 'all';
return $http.get(url)
.then(successCallback);
}
function getCurrentNews() {
var url = base + 'current';
return $http.get(url)
.then(successCallback);
}
function getNews(id) {
var url = base + id;
return $http.get(url)
.then(successCallback);
}
function updateNews(id, data, dataUrl) {
console.log('updateNews ', id);
var url = base + id;
if (dataUrl)
data.file = Upload.dataUrltoBlob(dataUrl, 'image.jpg');
return Upload.upload({
url: url,
data: data,
method: 'PUT'
});
}
function deleteNews(id) {
var url = base + id;
return $http.delete(url)
.then(successCallback);
}
function publishNews(id, publish) {
console.log('publishNews', id, publish);
var url = base + id + '/publish';
return $http.put(url, {},
{
params: {
'publish': publish
}
});
}
function adClicked(id) {
var url = base + 'seen/' + id;
return $http.put(url);
}
}
})();<file_sep>(function () {
'use strict';
angular
.module('app')
.directive('fileModel', fileModel);
/** @ngInject */
function fileModel() {
var directive = {
link: link,
restrict: 'A',
scope: {
fileModel: '='
}
};
return directive;
function link(scope, element) {
element.bind('change', change);
function change(event) {
var file = event.target.files[0];
scope.fileModel = file ? file : undefined;
scope.$apply();
}
}
}
})();
<file_sep>(function () {
'use strict';
angular
.module('app')
.controller('HomeController', HomeController);
/** @ngInject */
function HomeController($log, homeService, host, $timeout, categoryService, util, adService,
newsService, $scope, $window, applicationService) {
var vm = this;
vm.title = 'HomeController';
vm.dashboard = {};
vm.slides = [
{id: 0},
{id: 1},
{id: 2},
{id: 3},
{id: 4},
{id: 5},
{id: 6}
];
vm.host = host;
vm.colors = util.colors;
vm.getStarClass = getStarClass;
vm.getCategoryCol = getCategoryCol;
vm.seenAd = seenAd;
vm.myInterval = 3000;
vm.noWrapSlides = false;
vm.responsiveSetting = [
{
breakpoint: 964,
settings: {
slidesToShow: 2,
slidesToScroll: 2,
dots: true
}
},
{
breakpoint: 768,
settings: {
slidesToShow: 1,
slidesToScroll: 1,
dots: true
}
}
];
vm.downloadSimorgh = host + 'download/simorgh/ir.simorghmarket.simorgh.apk';
activate();
////////////////
function activate() {
$log.debug('Home module activated');
// getBanners();
//
// getSuggestedApps();
// getNewApps();
// getMostSeenApps();
// getBestApps();
//
// getCategories();
//
// getAds();
// getLatestNews();
getSimurgh();
}
function getSuggestedApps() {
homeService.getSuggestedApps().then(function (data) {
vm.suggestedApps = data;
$timeout(function () {
var width = $('.slick-slide').css("width");
/*if(width) {
width = parseInt(width.substring(0, width.length - 2));
console.log(width);
if (width < 80) {
$('.slick-slide').css("width", "80px")
}
}*/
}, 5000)
})
}
function getNewApps() {
homeService.getNewApps().then(function (data) {
vm.newApps = data;
/* $timeout(function () {
var width = $('.slick-slide').css("width");
width = parseInt(width.substring(0, width.length - 2));
console.log(width);
if (width < 80) {
$('.slick-slide').css("width", "80px")
}
}, 5000)*/
})
}
function getMostSeenApps() {
homeService.getMostSeenApps().then(function (data) {
vm.mostSeenApps = data;
})
}
function getBestApps() {
homeService.getBestApps().then(function (data) {
vm.bestApps = data;
})
}
function getStarClass(app, index) {
if (app.rating - index > 1) {
return "star";
}
else if (app.rating - index > 0) {
return "star-half-o";
} else {
return "star-o";
}
}
function getBanners() {
homeService.getPublishedBanners().then(function (data) {
vm.slides = data;
vm.active = 0;
});
}
function getCategories() {
categoryService.getAllCategories().then(function (data) {
vm.categories = data;
})
}
function getAds() {
adService.getCurrentAds().then(function (data) {
vm.ads = data;
})
}
function getLatestNews() {
newsService.getNewsList().then(function (data) {
vm.news = data.content;
})
}
function getCategoryCol(index, size) {
var len = vm.categories.length;
var i = index + (5 - i % 5);
var ii = index % 5;
if (len % 5 === 0 || index % 5 === 0 || i <= len) {
if (ii < 2)
return size === 6;
else if (ii > 1)
return size === 4;
} else {
var l = len % 5;
if (l === 1)
return size === 12;
if (l === 2)
return size === 6;
if (l === 3) {
if (ii === 1)
return size === 12;
else return size === 6;
}
if (l === 4)
return size === 6;
}
}
function seenAd(ad) {
adService.adClicked(ad.id);
window.open(ad.url, '_blank')
}
function getSimurgh() {
applicationService.getSimurgh().then(function (data) {
vm.simorghApp = data;
})
}
var w = angular.element($window);
$scope.$watch(function () {
return {
'h': w.height(),
'w': w.width()
};
}, function (newValue, oldValue) {
$scope.windowHeight = newValue.h;
$scope.windowWidth = newValue.w;
var c_width = $('#carousel-container').width();
var c_height = c_width * 300 / 880;
console.log(c_width, c_height);
$('#carousel-container').height(c_height);
$('.carousel-image').height(c_height);
$scope.resizeWithOffset = function (offsetH) {
$scope.$eval(attr.notifier);
return {
'height': (newValue.h - offsetH) + 'px'
//,'width': (newValue.w - 100) + 'px'
};
};
}, true);
w.bind('resize', function () {
$scope.$apply();
});
}
})();<file_sep>(function () {
'use strict';
angular
.module('app')
.factory('applicationService', applicationService);
/** @ngInject */
function applicationService($log, $http, host) {
var base = host + 'application/';
var service = {
getRecentApplications: getRecentApplications,
getSpecificApplications: getSpecificApplications,
getNumberOfApplications: getNumberOfApplications
};
return service;
////////////////
function getNumberOfApplications() {
var url = base + 'count';
return $http.get(url).then(successCallback);
}
function getSpecificApplications() {
var url = base + 'specific';
return $http.get(url).then(successCallback);
}
function getRecentApplications(size) {
var params = {
size: size || 20,
page: 0,
sort: 'createDate',
'createDate.dir': 'desc'
};
return $http.get(base, {params: params}).then(successCallback);
}
function successCallback(response) {
return response.data;
}
}
})();<file_sep>package ir.saafta.model;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import ir.saafta.dto.ApplicationStatus;
import org.springframework.context.annotation.Scope;
import javax.imageio.ImageIO;
import javax.persistence.*;
import java.awt.image.BufferedImage;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.Serializable;
import java.text.ParseException;
/**
* Created by reza on 11/13/16.
*/
@Entity
public class Picture implements Serializable {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private Long contentId;
@JsonIgnore
private byte[] data;
private String label;
private String type;
private String format;
private Boolean published;
private ApplicationStatus applicationStatus;
@Transient
private String url;
public Picture(byte[] data, String label, String type, String fileName) throws ParseException {
this.data = data;
this.label = label;
this.type = type;
this.published = false;
this.format = extractFormat(fileName);
this.applicationStatus = ApplicationStatus.PUBLIC;
}
public Picture(Long contentId, byte[] data, String label, String type, String fileNameOrFormat) throws ParseException {
this.contentId = contentId;
this.data = data;
this.label = label;
this.type = type;
this.published = false;
this.format = extractFormat(fileNameOrFormat);
this.applicationStatus = ApplicationStatus.PUBLIC;
}
public Picture(Long contentId, byte[] data, String label, String type, String fileNameOrFormat,
ApplicationStatus applicationStatus) throws ParseException {
this.contentId = contentId;
this.data = data;
this.label = label;
this.type = type;
this.published = false;
this.format = extractFormat(fileNameOrFormat);
this.applicationStatus = applicationStatus != null ? applicationStatus : ApplicationStatus.PUBLIC;
}
public Picture(){
}
private String extractFormat(String fileNameOrFormat) throws ParseException {
if (fileNameOrFormat == null)
throw new ParseException("Unknown file format", 0);
fileNameOrFormat = fileNameOrFormat.toLowerCase();
if (fileNameOrFormat.equals("jpg") || fileNameOrFormat.equals("jpeg"))
return "jpg";
else if (fileNameOrFormat.equals("png") || fileNameOrFormat.equals("tiff") ||
fileNameOrFormat.equals("gif") || fileNameOrFormat.equals("bmp"))
return fileNameOrFormat;
if (fileNameOrFormat.endsWith(".jpg") || fileNameOrFormat.endsWith(".jpeg"))
return "jpg";
else if (fileNameOrFormat.endsWith(".png"))
return "png";
else if (fileNameOrFormat.endsWith(".tiff"))
return "tiff";
else if (fileNameOrFormat.endsWith(".gif"))
return "gif";
else if (fileNameOrFormat.endsWith(".bmp"))
return "bmp";
else
throw new ParseException("Unknown file format", 0);
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public Long getContentId() {
return contentId;
}
public void setContentId(Long contentId) {
this.contentId = contentId;
}
public byte[] getData() {
return data;
}
public void setData(byte[] data) {
this.data = data;
}
public String getLabel() {
return label;
}
public void setLabel(String label) {
this.label = label;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getUrl() {
// String format = this.format != null ? this.format : "jpg";
String format = "jpg";
if (this.id != null) {
String url = "picture/" + this.id + "/pic";
if (this.contentId != null)
url += this.contentId + "_";
if (this.type != null)
url += this.type + "_";
url += this.id + "." + format;
return url;
}
return this.url;
}
public void setUrl(String url) {
this.url = url;
}
public Boolean getPublished() {
return published;
}
public void setPublished(Boolean published) {
this.published = published;
}
public String getFormat() {
return format;
}
public void setFormat(String format) throws ParseException {
this.format = extractFormat(format);
}
@Transient
public int getWidth() {
if (this.getData() == null)
return -1;
try {
InputStream in = new ByteArrayInputStream(this.getData());
BufferedImage image = ImageIO.read(in);
return image.getWidth();
} catch (Exception e) {
e.printStackTrace();
return 0;
}
}
@Transient
public int getHeight() {
if (this.getData() == null)
return -1;
try {
InputStream in = new ByteArrayInputStream(this.getData());
BufferedImage image = ImageIO.read(in);
return image.getHeight();
} catch (Exception e) {
e.printStackTrace();
return 0;
}
}
public ApplicationStatus getApplicationStatus() {
return applicationStatus;
}
public void setApplicationStatus(ApplicationStatus applicationStatus) {
this.applicationStatus = applicationStatus;
}
}
<file_sep>package ir.saafta.model;
import ir.saafta.dto.SessionStatus;
import javax.persistence.Entity;
import javax.persistence.EnumType;
import javax.persistence.Enumerated;
import javax.persistence.Id;
import java.util.Date;
/**
* Created by rkesh on 6/30/2017.
*/
@Entity
public class Session {
@Id
private String sessionId;
private String username;
private Date creationTime;
private Date finishTime;
@Enumerated(EnumType.ORDINAL)
private SessionStatus status;
private Date lastRequest;
private String remoteAddr;
private String deviceInfo;
public Session() {
}
public Session(String sessionId, String username, Date creationTime, Date finishTime, SessionStatus status, Date lastRequest, String remoteAddr, String deviceInfo) {
this.sessionId = sessionId;
this.username = username;
this.creationTime = creationTime;
this.finishTime = finishTime;
this.status = status;
this.lastRequest = lastRequest;
this.remoteAddr = remoteAddr;
this.deviceInfo = deviceInfo;
}
public String getSessionId() {
return sessionId;
}
public void setSessionId(String sessionId) {
this.sessionId = sessionId;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public Date getCreationTime() {
return creationTime;
}
public void setCreationTime(Date creationTime) {
this.creationTime = creationTime;
}
public Date getFinishTime() {
return finishTime;
}
public void setFinishTime(Date finishTime) {
this.finishTime = finishTime;
}
public SessionStatus getStatus() {
return status;
}
public void setStatus(SessionStatus status) {
this.status = status;
}
public Date getLastRequest() {
return lastRequest;
}
public void setLastRequest(Date lastRequest) {
this.lastRequest = lastRequest;
}
public String getRemoteAddr() {
return remoteAddr;
}
public void setRemoteAddr(String remoteAddr) {
this.remoteAddr = remoteAddr;
}
public String getDeviceInfo() {
return deviceInfo;
}
public void setDeviceInfo(String deviceInfo) {
this.deviceInfo = deviceInfo;
}
}
<file_sep>spring.datasource.url=jdbc:mysql://simorgh_db/simorgh?characterEncoding=utf-8
spring.datasource.username=suser
spring.datasource.password=<PASSWORD><file_sep>package ir.saafta.model;
import org.hibernate.annotations.FilterJoinTable;
import org.hibernate.annotations.WhereJoinTable;
import javax.persistence.*;
import java.util.List;
/**
* Created by rkesh on 7/4/2017.
*/
@Entity
@Table(name = "user")
public class UserProperties {
@Id
private String username;
@ManyToMany
@JoinTable(
name = "user_content",
joinColumns = @JoinColumn(name = "username", referencedColumnName = "username"),
inverseJoinColumns = @JoinColumn(name = "contentId", referencedColumnName = "id"))
@WhereJoinTable(clause = "bookmarked = true")
private List<Application> bookmarked;
@ManyToMany
@JoinTable(
name = "user_content",
joinColumns = @JoinColumn(name = "username", referencedColumnName = "username"),
inverseJoinColumns = @JoinColumn(name = "contentId", referencedColumnName = "id"))
@WhereJoinTable(clause = "install_date is not null")
private List<Application> downloaded;
@ManyToMany
@JoinTable(
name = "userContent",
joinColumns = @JoinColumn(name = "username", referencedColumnName = "username"),
inverseJoinColumns = @JoinColumn(name = "contentId", referencedColumnName = "id"))
@WhereJoinTable(clause = "bought = 1")
private List<Application> bought;
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public List<Application> getBookmarked() {
return bookmarked;
}
public void setBookmarked(List<Application> bookmarked) {
this.bookmarked = bookmarked;
}
public List<Application> getDownloaded() {
return downloaded;
}
public void setDownloaded(List<Application> downloaded) {
this.downloaded = downloaded;
}
public List<Application> getBought() {
return bought;
}
public void setBought(List<Application> bought) {
this.bought = bought;
}
}
| 986b71b1da7ed14a7666f384439c664f19a34127 | [
"SQL",
"JavaScript",
"Markdown",
"INI",
"Gradle",
"Java"
] | 109 | Java | rkeshmir/sbazaar | 56d11650481349e4e1ce65e949a21c86f4d5f01a | fd1117f57436615fc432ab7c73a64a3d96e00601 | |
HEAD | <file_sep>package ficha4;
import java.util.Map;
import java.util.TreeMap;
public class Gambiarra {
// atributos
private int numLampadas;
private boolean temEnergia;
// acessores
public int getNumLampadas() {
return numLampadas;
}
public void setNumLampadas(int numLampadas) {
this.numLampadas = numLampadas;
instalarLampadas();
}
public boolean isTemEnergia() {
return temEnergia;
}
public void setTemEnergia(boolean temEnergia) {
this.temEnergia = temEnergia;
}
// construtores
Gambiarra(int numLampadas) {
this.numLampadas = numLampadas;
instalarLampadas();
temEnergia = false;
}
Gambiarra() {
temEnergia = false;
}
// comportamentos
/*
* Solução para criar vários objetos com um ciclo tirado de:
* http://stackoverflow.com/questions/19590265
* /how-to-define-a-java-object-name-with-a-variable
*
* You seem to want to store and access objects using strings as a key. The
* way to do this is using a Map.
*
* Map<String,Cell> myMap = new TreeMap<String,Cell>();
*
* myMap.put("AA", new Cell(...));
* myMap.put("AB", new Cell(...));
*
* ...
*
* Cell currentCell = myMap.get("AA");
*
* Map is an interface. TreeMap is just one class that provides an
* implementation of the Map interface. Read up on the various
* implementations of Map provided by the standard JRE.
*
* The key doesn't have to be a String. Anything that implements equals()
* can be used as a key.
*
* Using this:
*
* for(char c = 'A'; c <= 'L'; c++) {
* for(char d = 'A'; d<= 'L'; d++) {
* myMap.put("" + c + d, new Cell(...));
* }
* }
*
* Minha interpretação:
* Generalização do comando: Map<String,Cell> myMap = new TreeMap<String,Cell>();
* Map<TipoDeDadoDeIdentificação,TipoDeDadoDeIdentificado(Objeto)>
* nomeDaVariavelDoMap =
* new TreeMap<TipoDeDadoDeIdentificação,Objeto>;
*
* Generalização do comando: myMap.put("" + c + d, new Cell(...));
* nomeDaVariavelDoMap.put(dadoDeIdentificação, new Objeto());
*
* Aqui queria usar um vetor do objeto Lampada, no entanto não o posso fazer
* diretamente. Ao usar Map posso criar o numero de lampadas que quizer e
* dar-lhes a referencia lampadaNumero1, lampadaNumero2, etc...
*/
//usa construtor por defeito (para testes)
private void instalarLampadas() {
Map<String, Lampada> lampadas = new TreeMap<String, Lampada>();
for (int i = 1; i <= numLampadas; i++) {
lampadas.put("lampadaNumero" + i, new Lampada());
}
}
private void instalarLampadas(int potencia) {
Map<String, Lampada> lampadas = new TreeMap<String, Lampada>();
for (int i = 1; i <= numLampadas; i++) {
lampadas.put("lampadaNumero" + i, new Lampada(potencia));
}
}
}
<file_sep>package ficha4;
public class Lampada {
//atributos
private int potencia;
private boolean acesa;
private boolean fundida;
//getters e setters
public boolean isAcesa () {
return acesa;
}
public int getPotencia() {
return potencia;
}
//construtores
Lampada (int potencia) {
this.potencia = potencia;
acesa = false;
fundida = false;
}
//construtor por defeito (para motivos de testes)
Lampada () {
potencia = 50;
acesa = false;
fundida = false;
}
//comportamentos
//alternativa mudar estado 1
//inicio alternatica 1
/* public void mudaEstado () {
* if (fundida == true) {
* acesa = false;
* } else {
* podeFundir();
* if (fundida == true) {
* //reportar que fundiu?
* acesa = false;
* } else acesa = !acesa;
* }
* }
*/
//fim alternativa1
//alternativa mudar estado 2
//inicio alternativa 1
public void mudaEstado() {
if (acesa == false) {
podeFundir();
if(fundida == false) {
podeMudarDeEstado();
}
} else podeMudarDeEstado();
}
private void podeMudarDeEstado () {
acesa = !acesa;
}
//fim alternativa 1
private void podeFundir () {
if (Math.random() <= 0.01) {
System.out.println("Uma lampada fundiu.");
fundida = true;
}
else
fundida = false;
}
}
| 04b75c1b99937d3988dbbf6b50c52ff88fb80f59 | [
"Java"
] | 2 | Java | JakkoPhD/aulasPOO-ficha4 | 7cea7cd81ad9c5fa2b2fe47ec341c000a719d967 | 9139ecf8b6e6e4d27654925b3a0c1f1a1c77dc8e | |
refs/heads/master | <file_sep>const mongoose = require('mongoose');
const smoothieSchema = mongoose.Schema({
title: { type: String, required: false },
ingredients: [
{nom: String, quantity: String},
],
features: {
cost: String ,
prepareTime: String
},
advice: String ,
description: String ,
steps: [
{ stepText: String } ,
],
photo: [
{ title: String, path: String, description: String}
]
/*
name: {
type: String,
required: false
},
photo: [
{
title: String,
path: String,
description: String
}
]
*/
});
const Smoothie = module.exports = mongoose.model('smoothie', smoothieSchema, );<file_sep>// catalogue des smoothie
var express = require('express');
var router = express.Router();
const Smoothie = require('../models/smoothie');
/* GET catalogue listing. */
router.get('/list', async function(req, res, next) {
/*
var smoothie = Smoothie.find({}, function(err, models) {
//var smoothie = Smoothie.find({"title": /fraise/i}, 'title id', function(err, models) {
if (err) {
res.render('error', {
status: 500
});
} else {
//res.json(models);
console.log(models);
res.send(models);
}
});
*/
try {
const smoothies = await Smoothie.find({});
res.send(smoothies);
} catch (err) {
console.log(err);
res.status(400).send(err);
}
});
router.get('/recipe/fruit/:fruit', async (req, res, next) => {
try {
let fruit = req.params.fruit;
const smoothies = await Smoothie.find({"title": new RegExp(fruit, 'i')});
//console.log('These are the recipes matched your request: ', smoothies);
res.send(smoothies);
} catch (err) {
console.log(err);
res.status(400).send(err);
}
})
router.get('/recipe/:id', async (req, res, next) => {
try {
const smoothies = await Smoothie.findById(req.params.id);
//console.log('These are the recipes matched your request: ', smoothies);
res.send(smoothies);
} catch (err) {
console.log(err);
res.status(400).send(err);
}
})
router.post('/recipe/add/', async function(req, res, next){
try {
console.log(req.body); // your JSON
const smoothie = await Smoothie.create(req.body);
response.send(smoothie); // echo the result back
} catch (err) {
console.log(err);
res.status(400).send(err);
}
});
module.exports = router;
| 2f1a2117d9caa629859023dbf69c1d0bfe7531eb | [
"JavaScript"
] | 2 | JavaScript | nguyenmk/smoothie | afed06bc79a4a5fa24df91096868e50173144d5b | 760327f8eefdc9cd3ea6b53fc74702f9d9e16bd5 | |
refs/heads/main | <file_sep>FROM golang:1.19-alpine as builder
COPY main.go .
ENV CGO_ENABLED=0
RUN go build -o /app main.go
FROM alpine
RUN apk add traceroute bind-tools curl
LABEL maintainer="github.com/bukowa"
# Define GOTRACEBACK to mark this container as using the Go language runtime
# for `skaffold debug` (https://skaffold.dev/docs/workflows/debug/).
ENV GOTRACEBACK=single
ENTRYPOINT ["./app"]
COPY --from=builder /app .
<file_sep>#!/bin/bash
trap "printf '\n' && trap - SIGTERM && kill -- -$$" SIGINT SIGTERM EXIT
set -x
# example docker:
# CMD="docker run --rm -i -p 9001:9001 <image> --port=9001" ./test.sh
exec 3< <(${CMD="go run . --port=9001"} 2>&1)
read <&3 line
set +x
if [[ $line =~ "Starting instance" ]]; then
echo $line
else
exit 1
fi
RESULT=$(curl -s -H 'User-Agent:' -H 'Header1: header1' -H 'Header2: header2' localhost:9001)
WANT='Instance name: example
Accept: [*/*]
Header1: [header1]
Header2: [header2]
Host: [localhost:9001]'
printf "===\nResult:\n===\n$RESULT\n"; printf "===\nWant:\n===\n$WANT\n===\n";
if [[ "$RESULT" != "$WANT" ]]; then
printf "test failed"; exit 1
else
echo "test passed"
fi
RESULT=$(curl -s -w "%{http_code}\n" "localhost:9001/livenessProbe")
if [[ "$RESULT" != 200 ]]; then
printf "test failed"; exit 1
else
echo "test passed"
fi
RESULT=$(curl -s -w "%{http_code}\n" "localhost:9001/readinessProbe")
if [[ "$RESULT" != 200 ]]; then
printf "test failed"; exit 1
else
echo "test passed"
fi
<file_sep>#!/bin/bash
set -eux
echo "Building docker image for git-cliff..."
GIT_CLIFF=$(docker build -q - <<EOF
FROM ghcr.io/orhun/git-cliff/git-cliff:sha-0f38960
WORKDIR /workdirvol
ENTRYPOINT ["git-cliff"]
EOF
)
echo "Running docker image for git-cliff..."
docker run --rm \
--volume="${PWD}:/workdirvol" \
-u "$(id -u)" \
"${GIT_CLIFF}" \
"$@"
<file_sep>module github.com/bukowa/http-headers
go 1.19
<file_sep>
generate-changelog:
./scripts/generate-changelog.sh
generate-changelog-ci:
touch CHANGELOG.md CHANGELOG.LATEST.md \
&& \
./scripts/generate-changelog.sh \
-u --prepend=CHANGELOG.md \
--include-path "*.go" \
--include-path "*.mod" \
--include-path "*Dockerfile" \
&& \
./scripts/generate-changelog.sh \
-u --output=CHANGELOG.LATEST.md \
--include-path "*.go" \
--include-path "*.mod" \
--include-path "*Dockerfile"
<file_sep>```bash
helm repo add bukowa https://bukowa.github.io/charts
helm install headers bukowa/http-headers
```
```bash
Instance name: example
Accept: [text/html,application/xhtml+xml,<cut>]
Accept-Encoding: [gzip, deflate]
Accept-Language: [en-US,en;q=0.5]
Host: [local.proxy]
Upgrade-Insecure-Requests: [1]
User-Agent: [Mozilla/5.0 <cut>]
X-Forwarded-For: [10.42.0.0]
X-Forwarded-Host: [http.mydev]
X-Forwarded-Port: [80]
X-Forwarded-Proto: [http]
X-Forwarded-Scheme: [http]
X-Real-Ip: [10.42.0.0]
X-Request-Id: [fadecae356e5fadf40b9b5b4419402ee]
X-Scheme: [http]
```
<file_sep># Changelog
All notable changes to this project will be documented in this file.
## [1.2.0]
### Features
- Add `traceroute` `bind-tools` `curl` | ( [ Commit ](https://github.com/bukowa/http-headers/commit/6ad464cebceba1936f200859279a2d2da4b22e7a) )
## [1.1.0]
### Features
- Add `livenessProbe` & `readinessProbe` ([#24](https://github.com/bukowa/http-headers/issues/24)) | ( [ Commit ](https://github.com/bukowa/http-headers/commit/a14d8fe9d03d039f28363196955c47807577908c) )
## [1.0.0]
### Core
- add docker to dependabot.yml
Signed-off-by: bukowa <<EMAIL>>
- add Host header manually ([#10](https://github.com/bukowa/http-headers/issues/10))
### Miscellaneous Tasks
- add new ci jobs for docker builds & changelogs ([#19](https://github.com/bukowa/http-headers/issues/19))
### Testing
- test new Dockerfile!
Signed-off-by: bukowa <<EMAIL>>
<file_sep># Changelog
All notable changes to this project will be documented in this file.
## [1.2.0]
### Features
- Add `traceroute` `bind-tools` `curl` | ( [ Commit ](https://github.com/bukowa/http-headers/commit/6ad464cebceba1936f200859279a2d2da4b22e7a) )
<!-- generated by git-cliff -->
<file_sep>package main
import (
"flag"
"fmt"
"log"
"net/http"
"sort"
"strings"
)
var Name = flag.String("name", "example", "name of instance")
func headers(w http.ResponseWriter, req *http.Request) {
log.Println("Hello! There's a request incoming!")
log.Printf("%v %v %v %v", req.RemoteAddr, req.URL, req.Host, req.Header)
// For incoming requests, the Host header is promoted to the
// Request.Host field and removed from the Header map.
req.Header.Set("host", req.Host)
sortedKeys := make([]string, 0, len(req.Header))
fmt.Fprintf(w, "Instance name: %s\n", *Name)
for name, _ := range req.Header {
sortedKeys = append(sortedKeys, name)
}
sort.Strings(sortedKeys)
for _, v := range sortedKeys {
fmt.Fprintf(w, "%v: %v\n", v, req.Header[v])
}
}
func livenessProbe(w http.ResponseWriter, req *http.Request) {
w.WriteHeader(200)
}
func readinessProbe(w http.ResponseWriter, req *http.Request) {
w.WriteHeader(200)
}
func main() {
http.HandleFunc("/livenessProbe", livenessProbe)
http.HandleFunc("/readinessProbe", readinessProbe)
http.HandleFunc("/", headers)
var host = flag.String("host", "", "host to run on")
var port = flag.String("port", "8080", "port to run on")
flag.Parse()
var address = strings.Join([]string{*host, *port}, ":")
log.Printf("Starting instance %s server at %s", *Name, address)
http.ListenAndServe(address, nil)
}
| 76bd2c77d96e64e7f7ee2241d2461871710c0353 | [
"Markdown",
"Makefile",
"Go",
"Go Module",
"Dockerfile",
"Shell"
] | 9 | Dockerfile | bukowa/golang-http-headers | 37a857038f58783c7b6d11e395f88f2fc72269dd | d946abf5afa5454412b9af5d9c294ed173695a8a | |
refs/heads/master | <repo_name>tom3197111/RWD_pages<file_sep>/index.php
<!DOCTYPE html>
<html lang="zh-Hant-TW">
<?php include('views/layouts/common.php'); ?>
<body class="terms" id="page-top " >
<!-- Navigation-->
<?php include('views/navbar/nav_common.php'); ?>
<!-- Masthead-->
<?php include('views/home/movie.php'); ?>
<?php include('views/Introduction/history.php'); ?>
<?php include('views/Introduction/produce.php'); ?>
<!-- About-->
<?php include('views/home/section1.php'); ?>
<!-- Services-->
<?php include('views/home/section2.php'); ?>
<!-- Portfolio-->
<?php include('views/home/section3.php'); ?>
<!-- Call to action-->
<?php include('views/home/section4.php'); ?>
<!-- Contact-->
<?php include('views/home/section5.php'); ?>
<!-- Footer-->
<?php include('views/layouts/common_footer.php'); ?>
<?php include('views/layouts/common_button.php'); ?>
<!-- Bootstrap core JS-->
<!-- Third party plugin JS-->
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery-easing/1.4.1/jquery.easing.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/magnific-popup.js/1.1.0/jquery.magnific-popup.min.js"></script>
<!-- Core theme JS-->
<script src="views/assets/js/scripts.js"></script>
</body>
<script>
//<![CDATA[
/*執行時間軸*/
$(".VivaTimeline").vivaTimeline();
//]]>
</script>
</html>
<file_sep>/views/assets/time_js/jquery.timelinr.js
(function(e, b, a, f) {
var d = "vivaTimeline";
var c = function(h, g) {
this.target = h;
this.carouselInterval;
this.checkImgLoad;
this.imgLoad = false;
this._init(g);
this._event()
};
c.options = {
carousel: true,
carouselTime: 10000
};
c.prototype = {
_init: function(h) {
var g = this;
g.options = e.extend(true, {}, c.options, h);
g.target.find(".events-body").each(function() {
var l = e(this).find(".row").length;
if (l > 1) {
var k = "<ol>";
for (var j = 0; j < l; j++) {
k += "<li data-target='" + j + "'></li>"
}
k += "</ol>";
e(this).siblings(".events-footer").html(k).find("li").first().addClass("active")
}
});
g.target.find(".events-body").each(function() {
e(this).find(".row").first().show().siblings().hide()
});
g.target.find("img").on("load", function() {
g.target.find(".events-body").each(function() {
var i = 0;
e(this).find(".row").each(function() {
if (e(this).height() > i) {
i = e(this).height()
}
});
e(this).find(".row").height(i)
})
})
},
_event: function() {
var g = this;
g.target.find(".events-header").click(function() {
e(this).siblings(".events-body").slideToggle().end().siblings(".events-footer").toggle()
});
g.target.find(".events-footer li").click(function() {
g._carousel(e(this))
});
if (g.options.carousel) {
g.carouselInterval = setInterval(function() {
g._carousel()
}, g.options.carouselTime);
g.target.find(".events").hover(function() {
clearInterval(g.carouselInterval);
g.carouselInterval = null
}, function() {
if (g.carouselInterval == f) {
g.carouselInterval = setInterval(function() {
g._carousel()
}, g.options.carouselTime)
}
})
}
},
_carousel: function(h) {
var g = this;
if (h == f) {
g.target.find(".events-footer .active").each(function() {
var j;
if (e(this).is(":last-child")) {
j = e(this).siblings().first()
} else {
j = e(this).next()
}
g._carousel(j)
})
} else {
var i = h.data().target;
h.addClass("active").siblings().removeClass("active");
h.closest(".events-footer").siblings(".events-body").find(".row").eq(i).show().siblings().hide()
}
}
};
e.fn[d] = function(h, g) {
var i;
this.each(function() {
i = new c(e(this), h)
});
return this
}
})(jQuery, window, document);<file_sep>/views/assets/js/scripts.js
/*!
* Start Bootstrap - Creative v6.0.3 (https://startbootstrap.com/themes/creative)
* Copyright 2013-2020 Start Bootstrap
* Licensed under MIT (https://github.com/StartBootstrap/startbootstrap-creative/blob/master/LICENSE)
*/
(function($) {
"use strict"; // Start of use strict
// Smooth scrolling using jQuery easing
$('a.js-scroll-trigger[href*="#"]:not([href="#"])').click(function() {
if (location.pathname.replace(/^\//, '') == this.pathname.replace(/^\//, '') && location.hostname == this.hostname) {
var target = $(this.hash);
target = target.length ? target : $('[name=' + this.hash.slice(1) + ']');
if (target.length) {
$('html, body').animate({
scrollTop: (target.offset().top - 72)
}, 1000, "easeInOutExpo");
return false;
}
}
});
// Closes responsive menu when a scroll trigger link is clicked
$('.js-scroll-trigger').click(function() {
$('.navbar-collapse').collapse('hide');
});
// Activate scrollspy to add active class to navbar items on scroll
$('body').scrollspy({
target: '#mainNav',
offset: 75
});
// Collapse Navbar
var navbarCollapse = function() {
if ($("#mainNav").offset().top > 100) {
$("#mainNav").addClass("navbar-scrolled");
} else {
$("#mainNav").removeClass("navbar-scrolled");
}
};
// Collapse now if page is not at top
navbarCollapse();
// Collapse the navbar when page is scrolled
$(window).scroll(navbarCollapse);
// Magnific popup calls
$('#portfolio').magnificPopup({
delegate: 'a',
type: 'image',
tLoading: 'Loading image #%curr%...',
mainClass: 'mfp-img-mobile',
gallery: {
enabled: true,
navigateByImgClick: true,
preload: [0, 1]
},
image: {
tError: '<a href="%url%">The image #%curr%</a> could not be loaded.'
}
});
})(jQuery); // End of use strict
//禁用F5
// $(document).ready(function(){ $(document).bind("keydown",function(e){var e=window.event||e;if(e.keyCode==116){ e.keyCode = 0; return false;} }) })
$(function() {
function isMobile() {
try{ document.createEvent("TouchEvent"); return true; }
catch(e){ return false;}
}
if(isMobile()){
$('.nav-link').css("padding","0.5rem 1rem")
$('.nav-link').css("display","block")
$('.dropdown-toggle').removeClass('dropdown-toggle')
$("#logo").width('250PX');
$(window).scroll(function() {
var scroHei = $(window).scrollTop();//滾動的高度
// alert(scroHei)
if (scroHei >= 1) {
$('.J_goTop').hide();
}if (scroHei >= 90) {
$('.J_goTop').show();
$('#two').fadeIn();
$('#two').addClass('animate__animated animate__backInRight');
}if(scroHei >= 680){
$('#three').fadeIn();
$('#three').addClass('animate__animated animate__backInDown');
}if(scroHei >= 1800){
$('#four').fadeIn();
$('#four').addClass('animate__animated animate__backInUp');
}if(scroHei >= 3620){
$('#five').fadeIn();
$('#five').addClass('animate__animated animate__backInDown');
}if(scroHei >= 4100){
$('#six').fadeIn();
$('#six').addClass('animate__animated animate__backInLeft');
}
else if(scroHei < 680){
$('#three').fadeOut();
}else if(scroHei < 1800){
$('#four').fadeOut();
}else if(scroHei < 3620){
$('#five').fadeOut();
}else if(scroHei < 4100){
$('#six').fadeOut();
}
})
}
else{ //scroll 事件適用於所有可滾動的元素和 window 對象(瀏覽器窗口)。
$(window).scroll(function() {
var scroHei = $(window).scrollTop();//滾動的高度
if (scroHei >= 1) {
$('.J_goTop').hide();
}if (scroHei >= 90) {
$('.J_goTop').show();
$('#two').fadeIn();
$('#two').addClass('animate__animated animate__backInRight');
}if(scroHei >= 600){
$('#three').fadeIn();
$('#three').addClass('animate__animated animate__backInDown');
}if(scroHei >= 1100){
$('#four').fadeIn();
$('#four').addClass('animate__animated animate__backInUp');
}if(scroHei >= 1700){
$('#five').fadeIn();
$('#five').addClass('animate__animated animate__backInDown');
}if(scroHei >= 2000){
$('#six').fadeIn();
$('#six').addClass('animate__animated animate__backInLeft');
}else if(scroHei < 90){
$('#two').fadeOut();
$('#three').fadeOut();
$('#four').fadeOut();
$('#five').fadeOut();
$('#six').fadeOut();
}else if(scroHei < 600){
$('#three').fadeOut();
}else if(scroHei < 1100){
$('#four').fadeOut();
}else if(scroHei < 1700){
$('#five').fadeOut();
}else if(scroHei < 2000){
$('#six').fadeOut();
}
})
}
/*點擊返回頂部*/
$('.J_goTop').click(function() {
$('body,html').animate({
scrollTop: 0
}, 600);
})
// window.onbeforeunload = function (evt) {
// var message = 'Are you sure you want to leave?';
// if (typeof evt == 'undefined') {
// evt = window.event;
// }
// if (evt) {
// evt.returnValue = message;
// }
// return message;
// }
$('#Introduction').click(function() {
$('body,html').animate({scrollTop: 0 }, 600);
$('#movie').removeClass(' animate__backInLeft');
$('#movie').addClass(' animate__backOutLeft');
// $('#produce_animate').removeClass(' animate__backInLeft');
// $('#produce_animate').addClass(' animate__backOutLeft');
$('#history').addClass(' animate__backInLeft');
$('#produce_title').fadeOut();
$('#movie_title').fadeOut();
$('#tow_title').fadeOut();
$('#three_title').fadeOut();
$('#four').fadeOut();
$('#five_title').fadeOut();
$('#six').fadeOut();
$('#four').attr('id','four_null');
$('#six').attr('id','six_null');
$('#history_tutle').fadeIn();
})
$('#produce').click(function() {
$('body,html').animate({scrollTop: 0 }, 600);
$('#movie').removeClass(' animate__backInLeft');
$('#movie').addClass(' animate__backOutLeft');
// $('#history').removeClass(' animate__backInLeft');
// $('#history').addClass(' animate__backOutLeft');
$('#produce_animate').addClass(' animate__backInLeft');
$('#history_tutle').fadeOut();
$('#movie_title').fadeOut();
$('#tow_title').fadeOut();
$('#three_title').fadeOut();
$('#four').fadeOut();
$('#five_title').fadeOut();
$('#six').fadeOut();
$('#four').attr('id','four_null');
$('#six').attr('id','six_null');
$('#produce_title').fadeIn();
})
$('#index').click(function() {
$('#movie').addClass(' animate__backInLeft');
$('#movie').removeClass(' animate__backOutLeft');
$('#history_tutle').fadeOut();
$('#produce_title').fadeOut();
$('#movie_title').fadeIn();
$('#tow_title').fadeIn();
$('#three_title').fadeIn();
$('#four').fadeIn();
$('#five_title').fadeIn();
$('#six').fadeIn();
$('#four_null').attr('id','four');
$('#six_null').attr('id','six');
})
})
| 978ff873742ebcd2b33361b11236db0d0a354f2a | [
"JavaScript",
"PHP"
] | 3 | PHP | tom3197111/RWD_pages | fb8c9a0c07f2eb8c89a943ae1dc92704a5654c6a | bb1318f3ac6a0513f55707f66c69416153f9cbbe | |
refs/heads/main | <file_sep>package Fox_game;
import java.awt.Color;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.Image;
import java.util.ArrayList;
import javax.swing.ImageIcon;
import javax.swing.JPanel;
import Fox_audio.Audio;
import Fox_sprite.Alien;
import Fox_sprite.Calcul;
import Fox_sprite.Chiffre;
import Fox_sprite.Ship;
@SuppressWarnings("serial")
public class Scene extends JPanel {
private ImageIcon icoSpaceBg;
private Image imgSpaceBg1;
private Image imgSpaceBg2;
private int yFond1;
private int yFond2;
private int deltaYFond;
public Ship ship;
private int deltaXShip;
private int score;
private int level;
private Font police1;
private Font police2;
public ArrayList<Chiffre> tabTir;
public ArrayList<Calcul> tabCalcul;
public ArrayList<Alien> tabAlien;
//Constructeur de la Scene
public Scene(){
super();
this.yFond1 = 0;
this.yFond2 = -1024;
this.deltaYFond = 1;
this.score = 0;
this.level = 0;
ship = new Ship(265,570);
this.deltaXShip=0;
icoSpaceBg = new ImageIcon(getClass().getResource("/Sprite/Arriere-plan.png"));
this.imgSpaceBg1 = this.icoSpaceBg.getImage();
this.imgSpaceBg2 = this.icoSpaceBg.getImage();
this.setFocusable(true);
this.requestFocusInWindow();
this.addKeyListener(new Clavier());
Calcul.vitesse = 0.10d;
this.tabTir = new ArrayList<Chiffre>();
this.tabCalcul = new ArrayList<Calcul>();
this.tabAlien = new ArrayList<Alien>();
police1 = new Font("Arial", Font.PLAIN, 22);
police2 = new Font("Arial", Font.PLAIN, 38);
Thread chronoEcran = new Thread(new Chrono());
chronoEcran.start();
}
/****************************METHODE****************************/
//methode qui gere le deplacement de l'ecran
public void deplacementFond(){
this.yFond1 += this.deltaYFond;
this.yFond2 += this.deltaYFond;
if(this.yFond1 == 1024){
this.yFond1 = -1024;
}else if(this.yFond2 == 1024){
this.yFond2 = -1024;
}
}
//methode qui gere le deplacement du vaisseau
public void deplacementShip(){
ship.setX(ship.getX()+this.deltaXShip);
}
public void deplacementAlien(Graphics g2){
if(tabAlien.size()>0){
for(Alien alien : tabAlien){
if(alien.getX() <= alien.getMaxX() && alien.getRegarde()=="droite"){
alien.regarde("droite");
}else if(alien.getX() > alien.getMaxX() && alien.getRegarde()=="droite"){
alien.regarde("gauche");
}else if(alien.getX() < alien.getMinX() && alien.getRegarde()=="gauche"){
alien.regarde("droite");
}
if(alien.getRegarde() == "droite"){
alien.setX(alien.getX()+1);
}else{
alien.setX(alien.getX()-1);
}
alien.setY(alien.getY()+Calcul.vitesse);
g2.drawImage(alien.getImgAlien(), alien.getX(), (int) alien.getY(), null);
}
}
}
//methode qui gere le deplacement des nombres
public void deplacementChiffre(Graphics g2){
for(int i=0; i<this.tabTir.size();i++){
if(this.tabTir.get(i).getVisible()==true){
g2.setColor(this.tabTir.get(i).getCOLOR());
g2.drawString(""+this.tabTir.get(i).getNum(), this.tabTir.get(i).getX(), (int)this.tabTir.get(i).getY());
this.tabTir.get(i).setY(this.tabTir.get(i).getY()-1);
this.tabTir.get(i).sortieEcran();
}else{
this.tabTir.remove(this.tabTir.get(i));
}
}
}
public void collisions(){
for(Chiffre tir : tabTir){
for(Calcul calc : tabCalcul){
if(tir.proche(calc)){
if(tir.getNum() == calc.getChiffreMistere()){
this.tabCalcul.remove(calc);
this.tabTir.remove(tir);
this.tabAlien.remove(calc.getAlien());
Audio.playSound("/Audio/alienMort.wav");
this.score++;
return;
}
}
}
}
}
public void verifScore(){
int palier = this.score / 5;
int modulo = this.score % 5;
if(this.level < palier && modulo == 0){
this.level++;
Calcul.vitesse += 0.1d;
Audio.playSound("/Audio/levelUp.wav");
}
}
//methode qui gere le deplacement des calculs mathematique
public void deplacementCalcul(Graphics g2){
for(Calcul calcul : tabCalcul){
calcul.setY(calcul.getY()+calcul.getVitesse());
g2.setFont(police2);
g2.setColor(calcul.getColor());
g2.drawString(calcul.getStrCalcul(), calcul.getX(), (int)calcul.getY());
if(calcul.getY()>570){
this.ship.setMort(true);
this.repaint();
}
}
}
//methode qui redessine l'ecran
public void paintComponent(Graphics g){
super.paintComponent(g);
Graphics g2 = (Graphics2D)g;
if(this.ship.isMort()==false){
this.deplacementFond();
this.deplacementShip();
this.collisions();
this.verifScore();
g2.drawImage(this.imgSpaceBg1, 0, this.yFond1, null);
g2.drawImage(this.imgSpaceBg2, 0, this.yFond2, null);
g2.drawImage(ship.getImgShip(), ship.getX(),(int)ship.getY(), null);
g2.setFont(police1);
g2.setColor(Color.ORANGE);
g2.drawString("Score = "+this.score+" pts", 15, 660);
g2.setColor(Color.green);
g2.drawString("Niveau = "+this.level, 400, 660);
this.deplacementCalcul(g2);
this.deplacementAlien(g2);
if(this.tabTir.size() > 0){
g2.setFont(police2);
deplacementChiffre(g2);
}
}else{
Audio.playSound("/Audio/boom.wav");
g2.setFont(police2);
g2.setColor(Color.RED);
g2.drawString("Vous avez perdu !!!", 120, 300);
g2.setColor(Color.black);
g2.drawString("Votre score est de : "+this.score, 80, 400);
}
}
/****************************************************************/
//GETTERS
public int getYFond1() {return yFond1;}
public int getDeltaYFond() {return deltaYFond;}
public int getDeltaXShip() {return deltaXShip;}
//SETTERS
public void setYFond1(int yFond1) {this.yFond1 = yFond1;}
public void setDeltaYFond(int deltaYFond) {this.deltaYFond = deltaYFond;}
public void setDeltaXShip(int deltaXShip) {this.deltaXShip = deltaXShip;}
}
<file_sep>package Fox_audio;
import javax.sound.sampled.AudioInputStream;
import javax.sound.sampled.AudioSystem;
import javax.sound.sampled.Clip;
public class Audio {
private Clip sound;
public Audio(String son){
try {
AudioInputStream audio = AudioSystem.getAudioInputStream(getClass().getResource(son));
sound = AudioSystem.getClip();
sound.open(audio);
}catch (Exception e) {}
}
//Getters
public Clip getSound() {return sound;}
//Methodes
public void play(){sound.start();}
public void stop(){sound.stop();}
public static void playSound(String son){
Audio s = new Audio(son);
s.play();
}
}
<file_sep>package Fox_game;
import Fox_sprite.Calcul;
public class Chrono implements Runnable{
private final int PAUSE = 4; // temps de pause entre chaque boucle pour le rafraichissement de l'ecran
private int tempsCreationCalcul;
private int i;
public Chrono(){
this.i = 0;
this.tempsCreationCalcul = 2500;
}
@Override
public void run() {
while(Main.scene.ship.isMort()==false){
this.i += PAUSE;
if(this.i > this.tempsCreationCalcul){
System.out.println("ajout calcul");
Main.scene.tabCalcul.add(new Calcul());
this.i=0;
}
Main.scene.repaint();
try {
Thread.sleep(PAUSE);
} catch (InterruptedException e) {}
}
}
}
<file_sep>package Fox_game;
import javax.swing.JFrame;
public class Main {
public static Scene scene;
//methode qui lance le programme
public static void main(String[] args) {
//creation de la fenetre de jeu
JFrame fenetre = new JFrame("Math Invasion");
fenetre.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
fenetre.setSize(575, 700);
fenetre.setLocationRelativeTo(null);
fenetre.setResizable(false);
fenetre.setAlwaysOnTop(true);
scene = new Scene();
fenetre.setContentPane(scene);
fenetre.setVisible(true);
}
}
<file_sep>package Fox_sprite;
import java.awt.Image;
import javax.swing.ImageIcon;
public class Alien extends Sprite{
private ImageIcon icoAlienDroite;
private ImageIcon icoAlienGauche;
private Image imgAlien;
private int minX;
private int maxX;
private String regarde;
private double vitesseDescente;
public Alien(int x, double y, int depLong, double vitesseDescente) {
super(62, 45, x, y);
this.icoAlienDroite = new ImageIcon(getClass().getResource("/Sprite/alienDroite.png"));
this.icoAlienGauche = new ImageIcon(getClass().getResource("/Sprite/alienGauche.png"));
this.setImgAlien(this.icoAlienDroite.getImage());
this.regarde = "droite";
this.minX = x;
this.maxX = x+depLong;
this.vitesseDescente = vitesseDescente;
}
public void regarde(String sens){
if(sens == "droite"){
this.setImgAlien(this.icoAlienDroite.getImage());
this.regarde = "droite";
}else if(sens == "gauche"){
this.setImgAlien(this.icoAlienGauche.getImage());
this.regarde = "gauche";
}
}
//GETTERS
public Image getImgAlien() {return imgAlien;}
public int getMinX() {return minX;}
public int getMaxX() {return maxX;}
public String getRegarde() {return regarde;}
public double getVitesseDescente() {return vitesseDescente;}
//SETTERS
public void setImgAlien(Image imgAlien) {this.imgAlien = imgAlien;}
}
<file_sep>package Fox_sprite;
import java.awt.Color;
import java.util.Random;
import Fox_game.Main;
public class Calcul extends Sprite{
private String strCalcul;
private Color color;
public static double vitesse;
private int chiffrePossible[] = {0,1,2,3,4,5,6,7,8,9};
private int chiffreMistere;
private Alien alien;
public Calcul() {
super(69, 400, 0, 50);
this.color = new Color(240,137,55);
this.strCalcul = this.creationFormule();
this.setLargeur(this.strCalcul.length()*18);
int xRand = this.randomEntreDeuxNombre(0, 575-this.getLargeur());
this.setX(xRand);
this.alien = new Alien(this.getX(),this.getY()-35, this.getLargeur(),this.getVitesse());
Main.scene.tabAlien.add(this.alien);
}
private String creationFormule(){
int i = randomEntreDeuxNombre(0, this.chiffrePossible.length);
int num1 = this.randomEntreDeuxNombre(1, 20);
this.chiffreMistere = this.chiffrePossible[i];
int res = num1 + this.chiffreMistere;
return num1+" + ? = "+res;
}
private int randomEntreDeuxNombre(int min, int max){
Random hasard = new Random();
int num = hasard.nextInt(max-min);
return num+min;
}
//GETTERS
public String getStrCalcul() {return strCalcul;}
public Color getColor() {return color;}
public double getVitesse() {return vitesse;}
public int getChiffreMistere() {return chiffreMistere;}
public Alien getAlien(){return this.alien;}
}
<file_sep># math_invasions
Jeu de calcul mental fait en JAVA. On deplace le vaisseau et on tire sur les extraterrestres avec les chiffres du pavé numerique pour resoudre le calcul.
 | 4961a7a2587d135f3000cd89e50edbefe5fca0bd | [
"Markdown",
"Java"
] | 7 | Java | NightFox26/math_invasions | 8e7504fc545eea79e6fdebb52ff899ec18c9ffe9 | 2107b84d6c0e3330bb217685b8165df38f42fab5 | |
HEAD | <file_sep>package ua.nure.tvshowmusic.db.dao.vote;
import org.springframework.stereotype.Repository;
import ua.nure.tvshowmusic.db.dao.AbstractCrudDao;
import ua.nure.tvshowmusic.model.Vote;
@Repository
public class DefaultVoteDao extends AbstractCrudDao<Vote> {
public DefaultVoteDao() {
super("vote");
}
}<file_sep>package ua.nure.tvshowmusic.db.dao.episode;
import org.springframework.jdbc.core.RowMapper;
import org.springframework.stereotype.Component;
import ua.nure.tvshowmusic.annotation.DefaultRowMapperQualifier;
import ua.nure.tvshowmusic.model.Episode;
import ua.nure.tvshowmusic.model.TvShow;
import java.sql.ResultSet;
import java.sql.SQLException;
@Component
@DefaultRowMapperQualifier
public class EpisodeRowMapper implements RowMapper<Episode> {
@Override
public Episode mapRow(ResultSet resultSet, int i) throws SQLException {
Episode episode = new Episode();
episode.setId(resultSet.getLong("id"));
TvShow tvShow = new TvShow();
tvShow.setId(resultSet.getLong("tv_show_id"));
episode.setTvShow(tvShow);
episode.setName(resultSet.getString("name"));
episode.setSeason(resultSet.getInt("season"));
episode.setNumber(resultSet.getInt("number"));
episode.setDate(resultSet.getDate("date"));
episode.setDescription(resultSet.getString("description"));
return episode;
}
}<file_sep>package ua.nure.tvshowmusic.exception;
public class SqlEntryExtractorException extends RuntimeException {
public SqlEntryExtractorException() {
}
public SqlEntryExtractorException(String message) {
super(message);
}
public SqlEntryExtractorException(String message, Throwable cause) {
super(message, cause);
}
public SqlEntryExtractorException(Throwable cause) {
super(cause);
}
}<file_sep>package ua.nure.tvshowmusic.db.entry.extractor;
import ua.nure.tvshowmusic.db.entry.SqlEntry;
import ua.nure.tvshowmusic.model.Model;
import java.util.List;
public interface DefaultSqlEntryExtractor<T> {
List<SqlEntry> extractSqlEntries(Model<T> model);
}<file_sep>db.driverClassName=com.mysql.cj.jdbc.Driver
db.url=jdbc:mysql://localhost:3306/tvshowmusic?useUnicode=true&useJDBCCompliantTimezoneShift=true&useLegacyDatetimeCode=false&serverTimezone=UTC
db.username=root
db.password=<PASSWORD><file_sep>package ua.nure.tvshowmusic.db.dao.user;
import org.springframework.jdbc.core.RowMapper;
import org.springframework.stereotype.Component;
import ua.nure.tvshowmusic.annotation.DefaultRowMapperQualifier;
import ua.nure.tvshowmusic.model.User;
import java.sql.ResultSet;
import java.sql.SQLException;
@Component
@DefaultRowMapperQualifier
public class UserRowMapper implements RowMapper<User> {
@Override
public User mapRow(ResultSet resultSet, int i) throws SQLException {
User user = new User();
user.setId(resultSet.getLong("id"));
user.setName(resultSet.getString("name"));
user.setEmail(resultSet.getString("email"));
user.setPassword(resultSet.getString("password"));
user.setAvatar(resultSet.getString("avatar"));
return user;
}
}<file_sep>package ua.nure.tvshowmusic.model;
import org.apache.commons.lang3.builder.ToStringBuilder;
import ua.nure.tvshowmusic.annotation.ManyToOne;
import java.io.Serializable;
import java.sql.Timestamp;
import java.util.Objects;
import static java.util.Objects.hash;
public class SubComment extends Model<Long> implements Serializable {
@ManyToOne
private User user;
@ManyToOne
private Comment comment;
private Timestamp date;
private String text;
public User getUser() {
return user;
}
public void setUser(User user) {
this.user = user;
}
public Comment getComment() {
return comment;
}
public void setComment(Comment comment) {
this.comment = comment;
}
public Timestamp getDate() {
return date;
}
public void setDate(Timestamp date) {
this.date = date;
}
public String getText() {
return text;
}
public void setText(String text) {
this.text = text;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SubComment that = (SubComment) o;
return Objects.equals(getId(), that.getId()) &&
Objects.equals(user, that.user) &&
Objects.equals(comment, that.comment) &&
Objects.equals(date, that.date) &&
Objects.equals(text, that.text);
}
@Override
public int hashCode() {
return hash(getId(), user, comment, date, text);
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("id", getId())
.append("user", user)
.append("comment", comment)
.append("date", date)
.append("text", text)
.toString();
}
}<file_sep>package ua.nure.tvshowmusic.model;
import org.apache.commons.lang3.builder.ToStringBuilder;
import ua.nure.tvshowmusic.annotation.ManyToOne;
import ua.nure.tvshowmusic.annotation.OneToMany;
import java.io.Serializable;
import java.sql.Date;
import java.util.List;
import java.util.Objects;
import static java.util.Objects.hash;
public class Episode extends Model<Long> implements Serializable {
@ManyToOne
private TvShow tvShow;
@OneToMany
private List<Appearance> appearances;
@OneToMany
private List<Comment> comments;
private String name;
private int season;
private int number;
private Date date;
private String description;
public TvShow getTvShow() {
return tvShow;
}
public void setTvShow(TvShow tvShow) {
this.tvShow = tvShow;
}
public List<Appearance> getAppearances() {
return appearances;
}
public void setAppearances(List<Appearance> appearances) {
this.appearances = appearances;
}
public List<Comment> getComments() {
return comments;
}
public void setComments(List<Comment> comments) {
this.comments = comments;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getSeason() {
return season;
}
public void setSeason(int season) {
this.season = season;
}
public int getNumber() {
return number;
}
public void setNumber(int number) {
this.number = number;
}
public Date getDate() {
return date;
}
public void setDate(Date date) {
this.date = date;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Episode episode = (Episode) o;
return Objects.equals(getId(), episode.getId()) &&
season == episode.season &&
number == episode.number &&
Objects.equals(tvShow, episode.tvShow) &&
Objects.equals(appearances, episode.appearances) &&
Objects.equals(comments, episode.comments) &&
Objects.equals(name, episode.name) &&
Objects.equals(date, episode.date) &&
Objects.equals(description, episode.description);
}
@Override
public int hashCode() {
return hash(getId(), tvShow, appearances, comments, name, season, number, date, description);
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("id", getId())
.append("tvShow", tvShow)
.append("appearances", appearances)
.append("comments", comments)
.append("name", name)
.append("season", season)
.append("number", number)
.append("date", date)
.append("description", description)
.toString();
}
}<file_sep>package ua.nure.tvshowmusic.db.dao.song;
import org.springframework.jdbc.core.RowMapper;
import org.springframework.stereotype.Component;
import ua.nure.tvshowmusic.annotation.DefaultRowMapperQualifier;
import ua.nure.tvshowmusic.model.Artist;
import ua.nure.tvshowmusic.model.Song;
import java.sql.ResultSet;
import java.sql.SQLException;
@Component
@DefaultRowMapperQualifier
public class SongRowMapper implements RowMapper<Song> {
@Override
public Song mapRow(ResultSet resultSet, int i) throws SQLException {
Song song = new Song();
song.setId(resultSet.getLong("id"));
Artist artist = new Artist();
artist.setId(resultSet.getLong("artist_id"));
song.setArtist(artist);
song.setName(resultSet.getString("name"));
song.setAlbum(resultSet.getString("album"));
song.setTime(resultSet.getInt("time"));
song.setArtworkUrl(resultSet.getString("artwork_url"));
song.setItunesUrl(resultSet.getString("itunes_url"));
song.setPreviewUrl(resultSet.getString("preview_url"));
return song;
}
}<file_sep>package ua.nure.tvshowmusic.db.dao.vote;
import ua.nure.tvshowmusic.db.dao.CrudDao;
import ua.nure.tvshowmusic.model.Vote;
public interface VoteDao extends CrudDao<Long, Vote> {
}<file_sep>package ua.nure.tvshowmusic.db.dao.appearance;
import org.springframework.stereotype.Repository;
import ua.nure.tvshowmusic.db.dao.AbstractCrudDao;
import ua.nure.tvshowmusic.model.Appearance;
@Repository
public class DefaultAppearanceDao extends AbstractCrudDao<Appearance> {
public DefaultAppearanceDao() {
super("appearance");
}
}<file_sep>package ua.nure.tvshowmusic.model;
import org.apache.commons.lang3.builder.ToStringBuilder;
import ua.nure.tvshowmusic.annotation.ManyToOne;
import ua.nure.tvshowmusic.annotation.OneToMany;
import java.io.Serializable;
import java.util.List;
import java.util.Objects;
import static java.util.Objects.hash;
public class Song extends Model<Long> implements Serializable {
@ManyToOne
private Artist artist;
@OneToMany
private List<Appearance> appearances;
private String name;
private String album;
private int time;
private String artworkUrl;
private String itunesUrl;
private String previewUrl;
public Artist getArtist() {
return artist;
}
public void setArtist(Artist artist) {
this.artist = artist;
}
public List<Appearance> getAppearances() {
return appearances;
}
public void setAppearances(List<Appearance> appearances) {
this.appearances = appearances;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAlbum() {
return album;
}
public void setAlbum(String album) {
this.album = album;
}
public int getTime() {
return time;
}
public void setTime(int time) {
this.time = time;
}
public String getArtworkUrl() {
return artworkUrl;
}
public void setArtworkUrl(String artworkUrl) {
this.artworkUrl = artworkUrl;
}
public String getItunesUrl() {
return itunesUrl;
}
public void setItunesUrl(String itunesUrl) {
this.itunesUrl = itunesUrl;
}
public String getPreviewUrl() {
return previewUrl;
}
public void setPreviewUrl(String previewUrl) {
this.previewUrl = previewUrl;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Song song = (Song) o;
return Objects.equals(getId(), song.getId()) &&
time == song.time &&
Objects.equals(artist, song.artist) &&
Objects.equals(appearances, song.appearances) &&
Objects.equals(name, song.name) &&
Objects.equals(album, song.album) &&
Objects.equals(artworkUrl, song.artworkUrl) &&
Objects.equals(itunesUrl, song.itunesUrl) &&
Objects.equals(previewUrl, song.previewUrl);
}
@Override
public int hashCode() {
return hash(getId(), artist, appearances, name, album, time, artworkUrl, itunesUrl, previewUrl);
}
@Override
public String toString() {
return new ToStringBuilder(this)
.append("id", getId())
.append("artist", artist)
.append("appearances", appearances)
.append("name", name)
.append("album", album)
.append("time", time)
.append("artworkUrl", artworkUrl)
.append("itunesUrl", itunesUrl)
.append("previewUrl", previewUrl)
.toString();
}
}<file_sep>package ua.nure.tvshowmusic.db.dao.comment;
import org.springframework.stereotype.Repository;
import ua.nure.tvshowmusic.db.dao.AbstractCrudDao;
import ua.nure.tvshowmusic.model.Comment;
@Repository
public class DefaultCommentDao extends AbstractCrudDao<Comment> {
public DefaultCommentDao() {
super("comment");
}
}<file_sep>package ua.nure.tvshowmusic.db.jdbc.template;
import org.springframework.jdbc.core.JdbcOperations;
import org.springframework.jdbc.core.RowMapper;
public interface ExtendedJdbcOperations extends JdbcOperations {
<T> T queryForObject(String sql, RowMapper<T> rowMapper, Object... args);
}<file_sep>package ua.nure.tvshowmusic.web.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import static org.springframework.web.bind.annotation.RequestMethod.GET;
@Controller
@RequestMapping("/logIn")
public class LogInController {
@RequestMapping(method = GET)
public String logIn() {
return "logIn";
}
}<file_sep>package ua.nure.tvshowmusic.db.dao.user;
import ua.nure.tvshowmusic.db.dao.CrudDao;
import ua.nure.tvshowmusic.model.User;
public interface UserDao extends CrudDao<Long, User> {
User read(String email);
}<file_sep>package ua.nure.tvshowmusic.db.dao.appearance;
import org.springframework.jdbc.core.RowMapper;
import org.springframework.stereotype.Component;
import ua.nure.tvshowmusic.annotation.DefaultRowMapperQualifier;
import ua.nure.tvshowmusic.model.Appearance;
import ua.nure.tvshowmusic.model.Episode;
import ua.nure.tvshowmusic.model.Song;
import java.sql.ResultSet;
import java.sql.SQLException;
import static ua.nure.tvshowmusic.model.Appearance.AppearanceStatus.valueOf;
@Component
@DefaultRowMapperQualifier
public class AppearanceRowMapper implements RowMapper<Appearance> {
@Override
public Appearance mapRow(ResultSet resultSet, int i) throws SQLException {
Appearance appearance = new Appearance();
appearance.setId(resultSet.getLong("id"));
Song song = new Song();
song.setId(resultSet.getLong("song_id"));
appearance.setSong(song);
Episode episode = new Episode();
episode.setId(resultSet.getLong("episode_id"));
appearance.setEpisode(episode);
appearance.setStatus(valueOf(resultSet.getString("status")));
appearance.setDescription(resultSet.getString("description"));
return appearance;
}
}<file_sep>package ua.nure.tvshowmusic.db.dao.artist;
import org.springframework.jdbc.core.RowMapper;
import org.springframework.stereotype.Component;
import ua.nure.tvshowmusic.annotation.DefaultRowMapperQualifier;
import ua.nure.tvshowmusic.model.Artist;
import java.sql.ResultSet;
import java.sql.SQLException;
@Component
@DefaultRowMapperQualifier
public class ArtistRowMapper implements RowMapper<Artist> {
@Override
public Artist mapRow(ResultSet resultSet, int i) throws SQLException {
Artist artist = new Artist();
artist.setId(resultSet.getLong("id"));
artist.setName(resultSet.getString("name"));
return artist;
}
} | d8b453f9c3442c11d226f5e690fa1091f988041e | [
"Java",
"INI"
] | 18 | Java | MaximRad/TvShowMusic | 253863d31c6ecceda0b32f848e02fdac35521d15 | 9a584ecb493140254700f9291da9b650a9364b08 | |
refs/heads/main | <repo_name>Thomas-roulland/CRUD-php<file_sep>/php/update.php
<?php
require_once "../BDD/db.php";
require_once "function.php";
include "../StructurePage/header.php";
$page=$_GET['page'];
if($page==1)
{
if(isset($_POST['id']))
{
$id = $_POST['id'];
$sql=Update("Organization",$_POST);
$db=connect('groups');
if($st=$db->prepare($sql))
{
var_dump($st->execute($_POST));
header('location:../index.php?page=1');
}
}
$resultat = "";
if (isset($_GET['id']))
{
$id = $_GET['id'];
// write SQL to get user data
//Execute the sql
$resultat = connect('groups')->query("SELECT * FROM Organization WHERE id='$id'");
$data = $resultat->fetch();
}
?>
<div class="text-light fs-1 text fw-bold text-center mb-5">
CRUD
</div>
<form action="update.php?id=<?php echo $id;?>&page=1" method="post" class="text-light">
<fieldset>
<legend>Mise à jour d'une organisation :</legend>
<label for="exampleInputPassword1" class="form-label">Nom : *</label>
<input type="text" class="form-control w-25 mb-2" name="name" value="<?php echo $data['name']; ?>">
<input type="hidden" name="id" value="<?php echo $id; ?>">
<label for="exampleInputPassword1" class="form-label">Domaine :</label>
<input type="text" class="form-control w-25 mb-2" name="domain" value="<?php echo $data['domain']; ?>">
<label for="exampleInputPassword1" class="form-label">Site :</label>
<input type="text" class="form-control w-25 mb-2" name="aliases" value="<?php echo $data['aliases']; ?>">
<br><br>
<button class="btn btn-primary" type="submit">Mettre à jour</button>
</fieldset>
</form>
<?php
}else
{
if(isset($_POST['id']))
{
$id = $_POST['id'];
$sql=Update("User",$_POST);
$db=connect('groups');
if($st=$db->prepare($sql))
{
var_dump($st->execute($_POST));
header('location:../index.php?page=2');
}
}
if (isset($_GET['id']))
{
$id = $_GET['id'];
// write SQL to get user data
//Execute the sql
$resultat = connect('groups')->query("SELECT * FROM User WHERE id='$id'");
$data = $resultat->fetch();
}
?>
<form action="update.php?id=<?php echo $id;?>&page=2" method="post" class="text-light">
<fieldset>
<legend>Mise à jour d'un User :</legend>
<label for="exampleInputPassword1" class="form-label">Prénom : *</label>
<input type="text" class="form-control w-25 mb-2" name="firstname" value="<?php echo $data['firstname']; ?>">
<input type="hidden" name="id" value="<?php echo $id; ?>">
<label for="exampleInputPassword1" class="form-label">Nom :</label>
<input type="text" class="form-control w-25 mb-2" name="lastname" value="<?php echo $data['lastname']; ?>">
<label for="exampleInputPassword1" class="form-label">Mot de passe :</label>
<input type="text" class="form-control w-25 mb-2" name="password" value="<?php echo $data['password']; ?>">
<label for="exampleInputPassword1" class="form-label">Email :</label>
<input type="text" class="form-control w-25 mb-2" name="email" value="<?php echo $data['email']; ?>">
<label for="exampleInputPassword1" class="form-label">Organization : *</label>
<select class="form-select w-25" name="idOrganization">
<?php
$organisation = connect('groups')->query("SELECT * FROM Organization");
foreach($organisation as $row) { ?>
<option value="<?php echo $row['id'] ?>"><?php echo $row['name'] ?></option>
<?php } ?>
</select>
<br><br>
<button class="btn btn-primary" type="submit">Mettre à jour</button>
</fieldset>
</form>
<?php
}
include '../StructurePage/footer.php';
<file_sep>/README.md
# CRUD-main2
<file_sep>/BDD/db.php
<?php
function connect(string $dbname){
$dbo=null;
try {
$dbo = new PDO("mysql:host=127.0.0.1;dbname=$dbname;charset=UTF8", 'root', '');
$dbo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
return $dbo;
}catch(\PDOException $e){
return false;
}
}<file_sep>/php/function.php
<?php
function create($table_name, $fieldvalues) {
$cmd_keys = array_keys($fieldvalues);
$cmd_values = array_values($fieldvalues);
$res="INSERT INTO $table_name(";
$res.= \implode(',', $cmd_keys);
$res.= ") VALUES(";
array_walk($fieldvalues, function(&$v){$v = "'$v'";});
$res.= \implode(',', $fieldvalues);
$res.= ");";
return $res;
}
function Update ($table,$fieldvalues){
$res="UPDATE `$table` SET";
$parts=[];
foreach ($fieldvalues as $key => $value){
$parts[]="`$key`= :$key ";
}
$res.= \implode(',',$parts);
return $res." WHERE id=:id";
}
function Delete ($table, $id){
$res="DELETE FROM `$table`";
return $res." WHERE id=$id";
}
function Getpage ($table){
$res="SELECT * FROM `$table`";
return $res;
}
?><file_sep>/php/work.php
<?php
require_once "../BDD/db.php";
require_once "function.php";
$cmd_connect = connect('groups');
$cmd = create("Organization", $_POST);
if(isset($_POST['name']) and isset($_POST['domain']) and isset($_POST['aliases']) and !empty($_POST['name'])) {
try {
if(connect('groups')->exec($cmd) == 1) {
?> <h1 class='success'><i class='fas fa-check'></i> Organisation ajoutée <br></h1>
<?php }
} catch(\PDOException $e) {
?>
<h1 class='error'><i class='fas fa-times'></i> Impossible d'ajouter l'organisation</h1>
<?php }
}
$cmd_connect1 = connect('groups');
$cmd1 = create("User", $_POST);
if(isset($_POST['firstname']) and isset($_POST['lastname']) and isset($_POST['email']) and isset($_POST['password']) and isset($_POST['idOrganization']) and !empty($_POST['firstname'])) {
try {
if(connect('groups')->exec($cmd1) == 1) {
?> <h1 class='success'><i class='fas fa-check'></i> User ajoutée <br></h1>
<?php }
} catch(\PDOException $e) {
?>
<h1 class='error'><i class='fas fa-times'></i> Impossible d'ajouter l'organisation</h1>
<?php }
}
if($_GET['id'])
$id=$_GET['id'];
$cmd_connect1 = connect('user');
$cmd1 = Delete("user", $id);
try {
if(connect('groups')->exec($cmd1) == 1) {
?> <h1 class='success'><i class='fas fa-check'></i> Suppression réussi <br></h1>
<?php }
}catch(\PDOException $e) {
?>
<h1 class='error'><i class='fas fa-times'></i> Impossible de supprimer</h1>
<?php }
if($_GET['id'])
$id=$_GET['id'];
$cmd_connect1 = connect('groups');
$cmd1 = Delete("Organization", $id);
try {
if(connect('groups')->exec($cmd1) == 1) {
?> <h1 class='success'><i class='fas fa-check'></i> Suppression réussi <br></h1>
<?php }
}catch(\PDOException $e) {
?>
<h1 class='error'><i class='fas fa-times'></i> Impossible de supprimer</h1>
<?php }
$page=$_GET['page'];
if($page==1){
header('location:../index.php?page=1');
}else{
header('location:../index.php?page=2');
}
<file_sep>/index.php
<?php
require_once "./BDD/db.php";
include "StructurePage/header.php";
?>
<div class="text-light fs-1 text fw-bold text-center mb-5 ">
CRUD
</div>
<!-- Button trigger modal -->
<?php
if($_GET['page']==1){
$statement=connect('groups')->query("SELECT * FROM Organization");
?>
<div class="d-flex justify-content-end mx-5">
<button type="button" class="btn btn-primary mb-5" data-bs-toggle="modal" data-bs-target="#exampleModal">
Ajoutez une organisation
</button>
</div>
<div class="modal fade" id="exampleModal" tabindex="-1" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<form action="php/work.php?page=1" method="POST">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Ajoutez une organisation :</h5>
<button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button>
</div>
<div class="modal-body">
<div class="mb-3 w-75">
<label for="exampleInputPassword1" class="form-label">Nom : *</label>
<input type="text" class="form-control align-middle" name="name" required>
</div>
<div class="mb-3 w-75">
<label for="exampleInputPassword1" class="form-label">Domaine :</label>
<input type="text" class="form-control" name="domain">
</div>
<div class="mb-3 w-75">
<label for="exampleInputPassword1" class="form-label">Site :</label>
<input type="text" class="form-control" name="aliases">
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-bs-dismiss="modal">Fermer</button>
<button type="submit" class="btn btn-primary">Ajouter</button>
</div>
</form>
</div>
</div>
</div>
<button class="btn btn-warning" onclick="window.location.href ='index.php?page=2'">
Table User
</button>
<table class="table text-light p-5 mb-5" >
<legend class="text-light fw-bold text-decoration-underline d-flex justify-content-left mt-5"> Données de la table Organisation :</legend>
<thead>
<tr>
<th>Id</th>
<th>Nom</th>
<th>Domain</th>
<th>User</th>
<th class="text-center">Action</th>
</tr>
</thead>
<tbody>
<?php
foreach($statement as $row) {
?>
<tr>
<td><?php echo $row['id']; ?></td>
<td><?php echo $row['name']; ?></td>
<td><?php echo $row['domain']; ?></td>
<td><?php echo $row['aliases']; ?></td>
<td class="text-center">
<a class="btn btn-info mx-2" href="./php/update.php?id=<?php echo $row['id'];?>&page=1">Edit</a>
<a class="btn btn-danger mx-2" href="./php/work.php?id=<?php echo $row['id'];?>&page=1">Delete</a>
</td>
</tr>
<?php
}
?>
</tbody>
</table>
<?php
} else {
$statement=connect('groups')->query("SELECT * FROM User");
$organisation = connect('groups')->query("SELECT * FROM Organization");
?>
<div class="d-flex justify-content-end mx-5">
<button type="button" class="btn btn-primary mb-5" data-bs-toggle="modal" data-bs-target="#exampleModal">
Ajoutez un User
</button>
</div>
<!-- Modal -->
<div class="modal fade" id="exampleModal" tabindex="-1" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<form action="php/work.php?page=2" method="POST">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Ajoutez un user :</h5>
<button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button>
</div>
<div class="modal-body">
<div class="mb-3 w-75">
<label for="exampleInputPassword1" class="form-label">Prénom : *</label>
<input type="text" class="form-control align-middle" name="firstname" required>
</div>
<div class="mb-3 w-75">
<label for="exampleInputPassword1" class="form-label">nom : *</label>
<input type="text" class="form-control align-middle" name="lastname" required>
</div>
<div class="mb-3 w-75">
<label for="exampleInputPassword1" class="form-label">email : *</label>
<input type="email" class="form-control align-middle" name="email" required>
</div>
<div class="mb-3 w-75">
<label for="exampleInputPassword1" class="form-label">password : *</label>
<input type="password" class="form-control align-middle" name="password" required>
</div>
<div class="mb-3 w-75">
<label for="exampleInputPassword1" class="form-label">Organization : *</label>
<select class="form-select" name="idOrganization">
<?php foreach($organisation as $row) { ?>
<option value="<?php echo $row['id'] ?>"><?php echo $row['name'] ?></option>
<?php } ?>
</select>
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-bs-dismiss="modal">Fermer</button>
<button type="submit" class="btn btn-primary">Ajouter</button>
</div>
</form>
</div>
</div>
</div>
<button class="btn btn-warning" onclick="window.location.href ='index.php?page=1'">
Table Organisation
</button>
<table class="table text-light p-5 w-100 mb-5" >
<legend class="text-light fw-bold text-decoration-underline d-flex justify-content-left mt-5"> Données de la table User :</legend>
<thead>
<tr>
<th>id</th>
<th>Prénom</th>
<th>Nom</th>
<th>email</th>
<th>password</th>
<th>Suspended</th>
<th>IdOrganization</th>
<th class="text-center">Action</th>
</tr>
</thead>
<tbody>
<?php
foreach($statement as $row) {
?>
<tr>
<td><?php echo $row['id']; ?></td>
<td><?php echo $row['firstname']; ?></td>
<td><?php echo $row['lastname']; ?></td>
<td><?php echo $row['email']; ?></td>
<td><?php echo $row['password']; ?></td>
<td><?php echo $row['suspended']; ?></td>
<td><?php echo $row['idOrganization']; ?></td>
<td class="text-center">
<a class="btn btn-info mx-2" href="./php/update.php?id=<?php echo $row['id']; ?>&page=2">Edit</a>
<a class="btn btn-danger mx-2" href="./php/work.php?id=<?php echo $row['id']; ?>&page=2">Delete</a>
</td>
</tr>
<?php
}
} ?>
</tbody>
</table>
<?php include 'StructurePage/footer.php'; ?>
| 052ea9eae1bb9954a1619de1ca14672d19e15d3f | [
"Markdown",
"PHP"
] | 6 | PHP | Thomas-roulland/CRUD-php | 9f5b117af34dd74fb029ee3b165076e5dbdf0525 | 416a6ac32252ee31a5b351f4a9fe7c2585f7914d | |
refs/heads/master | <repo_name>princenishchal/tllr<file_sep>/src/pages/transdetail/transdetail.ts
import { Component, Input, ViewChild, ElementRef, Renderer2, NgZone, ChangeDetectorRef} from '@angular/core';
import { NavController, NavParams, AlertController, Platform, Events } from 'ionic-angular';
/**
* import pages here
*/
import {TransdetailChatPage} from './transdetail-chat/transdetail-chat';
import {SendLocationPage} from '../send-location/send-location'
import {SelectPhotosPage} from '../select-photos/select-photos';
import {ImagePreviewPage} from '../image-preview/image-preview';
import {Observable} from 'rxjs';
@Component({
selector: 'page-transdetail',
templateUrl: 'transdetail.html',
})
export class TransdetailPage {
// the main transaction details object.
transactionDetails:any = {
photos:null,
location:null,
friends:[],
chat:{}
}
chatPage = TransdetailChatPage;
chatParams = {};
@ViewChild('transDetailBlock') transDetailBlock:ElementRef;
@ViewChild('transDetailTags') transDetailTags:ElementRef;
@ViewChild('transDetailTagSpan') transDetailTagSpan:ElementRef;
@ViewChild('transDetailNotes') transDetailNotes:ElementRef;
@ViewChild('transDetailNotesSpan') transDetailNotesSpan:ElementRef;
@ViewChild('chatsDetailBlock') chatsDetailBlock:ElementRef;
@ViewChild('tagFriendsDivBlock') tagFriendsDivBlock:ElementRef;
constructor(private ref:ChangeDetectorRef, public navCtrl: NavController, public navParams: NavParams, public alertCtrl: AlertController, public renderer: Renderer2) {
}
ionViewDidLoad() {
console.log('ionViewDidLoad TransdetailPage');
}
// pushes the add photos page with a call back to do processing after the photos have been collected
selectPhotos(){
this.navCtrl.push(SelectPhotosPage,{
callback:(photos)=>{
return new Promise((resolve,reject)=>{
let photostoUpload = photos.map(p=>{
return p.blob
});
this.transactionDetails.photos = photos.map(p=> {
return p.src
});
//TODO: upload images and then show them in the images.
resolve();
this.ref.detectChanges();
})
}
})
}
// fetches a location object and shows it in the transaction details page
addLocation(){
this.navCtrl.push(SendLocationPage,{
callback:(location)=>{
return new Promise((resolve,reject)=>{
//TODO: uplaod the location and then add it to the local object
console.log(location)
this.transactionDetails.location = location;
resolve();
})
}
})
}
previewImages(images,startAt){
this.navCtrl.push(ImagePreviewPage,{
images:images,
startAt:startAt,
title:"chic-fill-a"
})
}
}
<file_sep>/src/providers/chat-service/chat-service.ts
import { Injectable } from '@angular/core';
import { Socket } from 'ng-socket-io';
import {Observable,Subject} from 'rxjs'
/*
Generated class for the ChatServiceProvider provider.
See https://angular.io/guide/dependency-injection for more info on providers
and Angular DI.
*/
@Injectable()
export class ChatServiceProvider {
private // messages queued in thread
private _newMessage:Subject<any>;
newMessage:Observable<any>;
constructor(private socket:Socket) {
console.log('Hello ChatServiceProvider Provider');
this._newMessage = new Subject<any>();
this.newMessage = this._newMessage.asObservable();
this.socket.on('message',(message)=>{
this._newMessage.next(message);
})
}
send(message){
//TODO; do something with the message object
this.socket.emit('message',message);
}
}
<file_sep>/src/pages/select-photos/select-photos.ts
import { Component, ChangeDetectorRef } from '@angular/core';
import { DomSanitizer } from '@angular/platform-browser';
import { NavController, NavParams } from 'ionic-angular';
declare var cordova: any;
import { PhotoLibrary, LibraryItem, GetLibraryOptions, } from '@ionic-native/photo-library';
import { Subject } from 'rxjs';
/**
* Generated class for the SelectPhotosPage page.
*
* See https://ionicframework.com/docs/components/#navigation for more info on
* Ionic pages and navigation.
*/
@Component({
selector: 'page-select-photos',
templateUrl: 'select-photos.html',
})
export class SelectPhotosPage {
images = [];
private gallaryImages = null;
private pauser: Subject<boolean>; // used for pausing the library sequesnce
private infiniteScrollHandle: any;
private page = 0;
private chunk = 30;
private callback: Function; // a pomise type function
listNotEmpty: boolean;
isLoading: boolean = true;
constructor(public navCtrl: NavController, public navParams: NavParams, private photoLibrary: PhotoLibrary, private changeRef: ChangeDetectorRef, private sanitizer: DomSanitizer) {
this.callback = this.navParams.data.callback;
/**
* TODO : remove this after testing
*/
/* this.images = new Array(100).fill({
creationDate:Date.now(),
thumbnailURL: "https://placeholdit.co//i/71x76?&bg=9b9b9b"
}).map((img,index,arr)=>{
img.index = index;
return img
}) */
this.listNotEmpty = false;
let opts: GetLibraryOptions = {};
opts.thumbnailHeight = 76;
opts.thumbnailWidth = 71;
opts.includeAlbumData = true;
this.photoLibrary.requestAuthorization().then(() => {
this.photoLibrary.getLibrary(opts).subscribe({
next: library => {
/* library.forEach(function(libraryItem) {
console.log(libraryItem.id); // ID of the photo
console.log(libraryItem.photoURL); // Cross-platform access to photo
console.log(libraryItem.thumbnailURL);// Cross-platform access to thumbnail
console.log(libraryItem.fileName);
console.log(libraryItem.width);
console.log(libraryItem.height);
console.log(libraryItem.creationDate);
console.log(libraryItem.latitude);
console.log(libraryItem.longitude);
console.log(libraryItem.albumIds); // array of ids of appropriate AlbumItem, only of includeAlbumsData was used
});*/
this.gallaryImages = library.sort(
(a: LibraryItem, b: LibraryItem) => {
return new Date(a.creationDate) >= new Date(b.creationDate) ? -1 : 1;
}
);
this.gallaryImages.slice(this.page * this.chunk, (this.page + 1) * this.chunk).map(img => {
let imjObj = {
thumbnailURL: this.sanitizer.bypassSecurityTrustUrl(img.thumbnailURL),
photoUrl:this.sanitizer.bypassSecurityTrustUrl(img.photoURL),
fileName: img.fileName,
id: img.id,
creationDate: img.creationDate,
index: this.images.length,
selected: false
}
// sanitize the urls before pushing
this.images.push(imjObj);
})
console.log(this.images);
this.page += 1;
this.isLoading = false;
this.changeRef.detectChanges();
},
error: err => { console.log('could not get photos'); },
complete: () => { console.log('done getting photos'); }
});
})
.catch(err => console.log('permissions weren\'t granted'));
}
doInfinite(infiniteScroll) {
this.gallaryImages.slice(this.page * this.chunk, (this.page + 1) * this.chunk).map(img => {
let imjObj = {
thumbnailURL: this.sanitizer.bypassSecurityTrustUrl(img.thumbnailURL),
fileName: img.fileName,
id: img.id,
creationDate: img.creationDate,
index: this.images.length
}
// sanitize the urls before pushing
this.images.push(imjObj);
})
infiniteScroll.complete();
this.page += 1;
this.changeRef.detectChanges();
}
shouldAddStamp(img) {
if (this.images.length >= 2 && img.index != 0)
return !this.sameDay(img.creationDate, this.images[img.index - 1].creationDate);
else
return true;
}
select(index) {
this.images[index].selected = true;
this.checkListEmpty();
this.changeRef.detectChanges();
}
unselect(index) {
this.images[index].selected = false;
this.checkListEmpty();
this.changeRef.detectChanges();
}
// get actual iamges
getPhotos() {
let selectedPhotos = this.images.filter(i => i.selected);
// fetch photos from the ionic native
Promise.all(
selectedPhotos.map(sp => {
return this.photoLibrary.getPhoto(sp.id)
})
).then(photos => {
// send back selected photos with local urls
let imagestoReurn = selectedPhotos.map((sp,i)=>{
return {
src: sp.photoUrl,
blob: photos[i]
};
})
console.log("photso fetched", imagestoReurn);
this.callback(imagestoReurn).then(() => {
this.navCtrl.pop()
})
})
}
private checkListEmpty() {
this.listNotEmpty = this.images.filter(i => i.selected).length ? true : false;
}
private sameDay(date1, date2) {
let d1 = new Date(date1);
let d2 = new Date(date2);
return d1.getFullYear() === d2.getFullYear() &&
d1.getMonth() === d2.getMonth() &&
d1.getDate() === d2.getDate();
}
ionViewDidLoad() {
console.log('ionViewDidLoad SelectPhotosPage');
}
}
<file_sep>/src/components/components.module.ts
import { NgModule } from '@angular/core';
import { CommonModule} from '@angular/common';
import { AvatarComponent } from './avatar/avatar';
import { PlacesAutoCompleteComponent } from './places-auto-complete/places-auto-complete';
import { IonicModule } from 'ionic-angular';
import { FormsModule } from '@angular/forms';
@NgModule({
declarations: [AvatarComponent,
PlacesAutoCompleteComponent],
imports: [CommonModule,FormsModule, IonicModule],
exports: [AvatarComponent,
PlacesAutoCompleteComponent]
})
export class ComponentsModule {}
<file_sep>/README.md
# tllr
# Setup:
1. clone the master branch
2. run npm install
3. run cordova plugins install
# Desccription:
Contains the location picker and select-contacts plugins.
# TRANSFERING COPONENTS AND SETUP
1.dependencies:
1. Make sure that package @ngui/map is installed ( for the maps component)
2. Make sure cordova-plugin-contacts-phonenumbers is installed via cordova plugin add cordova-plugin-contacts-phonenumbers
[https://github.com/dbaq/cordova-plugin-contacts-phone-numbers](link to plugin github)
2.Copying code:
1. From the app.module.ts file .. copy over the lines marked with /** COPY THIS */
2. From the app.scss file , copy over css marked with /** COPY THIS */
3. Copy the select-contacts and the location-picker folders into the destiantion , keeping the same hirarchy
# using the components
1. the location picker is invoked declaratively from a button , link etc via the navPush attribut. In this repo , you will find the example in the home component:
**<button ion-button [navPush]="pushPage" [navParams]="params">select location</button>**.
YOU MUST IMPORT THE locationPickerPageComponent in the page from where you are goign to invoke this.
2. when the locaiton picker is invoked and a location is selected , the location picker pushes the select contacts component , passing the selected address to it.
once the contacts are selected and the continue button is clicked , the **confirm()** function in the component collects the address and selected contacts for passing on. This function has been stubbed for now.
# Important
the contacts page will not work in the browser and will fail, please test it with emulation or a real device.
# Select Photos component
## plugins used:
1. ionic native photos - https://ionicframework.com/docs/native/photo-library/ . follow installation steps in the page
## copy contents
1. copy the select-photos folder and add component class to app.module.
## notes:
1. The getPhotos function returns an array of photo blobs .. These can be directly uploaded using formData.
2. The photos will not show up in the latest webview (Wk) .. when testing ensure that the app has the older webview .
# add-locaiton
1. copy contents of components folder
2. install ionic native geolocation , follow steps mentioned
3. add this config in xml
`<edit-config file="*-Info.plist" mode="merge" target="NSLocationWhenInUseUsageDescription">
<string>To get your location </string>
</edit-config>`<file_sep>/src/pages/image-preview/image-preview.ts
import { Component, ViewChild } from '@angular/core';
import { NavController, NavParams ,Slides} from 'ionic-angular';
import { Slide } from 'ionic-angular/components/slides/slide';
/**
* Generated class for the ImagePreviewPage page.
*
* See https://ionicframework.com/docs/components/#navigation for more info on
* Ionic pages and navigation.
*/
@Component({
selector: 'page-image-preview',
templateUrl: 'image-preview.html',
})
export class ImagePreviewPage {
images:any[];
private startAt:number;
title:string= "";
currentSlide:number;
@ViewChild('slider') slider :Slides;
constructor(public navCtrl: NavController, public navParams: NavParams) {
this.images = navParams.data.images;
this.startAt = navParams.data.startAt;
this.title = navParams.data.title;
this.currentSlide = this.startAt+1;
}
onSlideChange(val){
this.currentSlide = this.slider.getActiveIndex()+1;
}
}
<file_sep>/src/app/app.module.ts
import { BrowserModule } from '@angular/platform-browser';
import { ErrorHandler, NgModule } from '@angular/core';
import { IonicApp, IonicErrorHandler, IonicModule } from 'ionic-angular';
import { SplashScreen } from '@ionic-native/splash-screen';
import { StatusBar } from '@ionic-native/status-bar';
import { MyApp } from './app.component';
import { HomePage } from '../pages/home/home';
import { TransdetailPage } from '../pages/transdetail/transdetail';
/** COPY THIS */
import {LocationPickerPage} from '../pages/location-picker/location-picker';
import {SelectContactsPage} from '../pages/select-contacts/select-contacts'
import { NguiMapModule} from '@ngui/map';
import { EmojifyModule } from 'angular2-emojify';
import { TransdetailChatPage } from '../pages/transdetail/transdetail-chat/transdetail-chat';
import { ChatMessageComponent } from '../pages/transdetail/transdetail-chat/chat-message/chat-message';
import { SelectPhotosPage} from '../pages/select-photos/select-photos';
import {SendLocationPage} from '../pages/send-location/send-location';
import {ImagePreviewPage} from '../pages/image-preview/image-preview';
import { SocketIoModule, SocketIoConfig } from 'ng-socket-io';
import {MomentModule} from 'angular2-moment';
import {PhotoLibrary} from '@ionic-native/photo-library';
import { Geolocation } from '@ionic-native/geolocation';
/** import the components module here */
import {ComponentsModule} from '../components/components.module';
/** COPY this config for socet io */
const config: SocketIoConfig = { url: 'http://finterest.co:5500/', options: {} };
@NgModule({
declarations: [
MyApp,
HomePage,
LocationPickerPage,
SelectContactsPage,
TransdetailPage,
TransdetailChatPage,
ChatMessageComponent,
SelectPhotosPage,
SendLocationPage,
ImagePreviewPage
],
imports: [
BrowserModule,
IonicModule.forRoot(MyApp),
/** place api key here */
/** COPY THIS */
ComponentsModule,
MomentModule,
NguiMapModule.forRoot({apiUrl: 'https://maps.google.com/maps/api/js?v=3&key=<KEY>&libraries=places'}),
EmojifyModule,
SocketIoModule.forRoot(config)
],
bootstrap: [IonicApp],
entryComponents: [
MyApp,
HomePage,
/** COPY THIS */
LocationPickerPage,
SelectContactsPage,
TransdetailPage,
TransdetailChatPage,
ChatMessageComponent,
SelectPhotosPage,
SendLocationPage,
ImagePreviewPage
],
providers: [
StatusBar,
SplashScreen,
{provide: ErrorHandler, useClass: IonicErrorHandler},
PhotoLibrary,
Geolocation
]
})
export class AppModule {}
<file_sep>/src/pages/select-contacts/select-contacts.ts
import { Component, ChangeDetectorRef } from '@angular/core';
import { NavController, NavParams } from 'ionic-angular';
import { DomSanitizer } from '@angular/platform-browser';
declare var navigator: any;
/**
* Generated class for the SelectContactsPage page.
*
* See https://ionicframework.com/docs/components/#navigation for more info on
* Ionic pages and navigation.
*/
@Component({
selector: 'page-select-contacts',
templateUrl: 'select-contacts.html',
})
export class SelectContactsPage {
contacts: any[] = []
private address: any;
listNotEmpty: boolean = false;
private infiniteScrollHandle: any;
private allContacts: any[];
private searchResults:any[] = [];
private page = 0;
private chunk = 30;
search:string = null;
isLoading: boolean = true;
constructor(public navCtrl: NavController, public navParams: NavParams, private changeRef: ChangeDetectorRef, private domsanitizer: DomSanitizer) {
this.address = navParams.data; // just keep the data here
}
ionViewDidLoad() {
console.log('ionViewDidLoad SelectContactsPage');
if (navigator.contactsPhoneNumbers)
navigator.contactsPhoneNumbers.list((contacts: any[]) => {
this.contacts = [];
// sort in asc
this.allContacts = contacts.sort((a, b) => a.displayName >= b.displayName ? 1 : -1).map((ac,i)=>{
return {
idx: i,
id: ac.id,
picture: ac.thumbnail ? this.domsanitizer.bypassSecurityTrustUrl(ac.thumbnail) : null,
phone: ac.phoneNumbers[0].normalizedNumber,
name: ac.displayName,
selected: false
}
})
this.allContacts.slice(this.page * this.chunk, (this.page + 1) * this.chunk).map((contact, i) => {
this.contacts.push({
idx: contact.idx,
idy: i,
id: contact.id,
picture: contact.thumbnail ? this.domsanitizer.bypassSecurityTrustUrl(contact.thumbnail) : null,
phone: contact.phone,
name: contact.name,
selected: contact.selected
});
})
this.page += 1;
this.isLoading = false;
this.changeRef.detectChanges();
this.changeRef.detectChanges();
}, (error) => {
console.error(error);
});
}
selectContact(idx,idy) {
this.allContacts[idx].selected = true;
if( idy && this.contacts[idy]) this.contacts[idy].selected = true;
else{
this.contacts.map((c,i)=>{
if(c.idx == idx) this.contacts[i].selected = true;
})
}
this.isListEmpty()
this.changeRef.detectChanges();
}
removeContact(idx,idy) {
this.allContacts[idx].selected = false;
if( idy && this.contacts[idy]) this.contacts[idy].selected = false;
else{
this.contacts.map((c,i)=>{
if(c.idx == idx) this.contacts[i].selected = false;
})
}
this.isListEmpty();
this.changeRef.detectChanges();
}
isListEmpty() {
this.listNotEmpty = this.contacts.filter(c => c.selected).length ? true : false;
}
confirm() {
// do something with the selected contacts and address
let dataToSubmit = {
selectedContacts: this.contacts.filter(c => c.selected == true),
selectedAddress: this.address
}
console.log(dataToSubmit);
this.navCtrl.popToRoot();
}
doInfinite(infiniteScroll) {
this.allContacts.slice(this.page * this.chunk, (this.page + 1) * this.chunk).map((contact, i) => {
this.contacts.push({
idx: contact.idx,
idy:i,
id: contact.id,
picture: contact.thumbnail ? this.domsanitizer.bypassSecurityTrustUrl(contact.thumbnail) : null,
phone: contact.phone,
name: contact.name,
selected: contact.selected
});
})
infiniteScroll.complete();
this.page += 1;
this.changeRef.detectChanges();
}
searchEmployee(name){
this.searchResults = name? this.allContacts.filter(c=>c.name.indexOf(name) > -1) || [] : [];
}
addFromSearch(contact){
this.searchResults = [];
this.search = null;
// mark as selected in all contacts
this.selectContact(contact.idx, contact.idy || null)
}
}
<file_sep>/src/pages/home/home.ts
import { Component } from '@angular/core';
import { NavController } from 'ionic-angular';
import {LocationPickerPage} from '../location-picker/location-picker';
import { TransdetailPage } from '../transdetail/transdetail';
import {SelectPhotosPage} from '../select-photos/select-photos';
import {SendLocationPage} from '../send-location/send-location';
import {SelectContactsPage} from '../select-contacts/select-contacts';
@Component({
selector: 'page-home',
templateUrl: 'home.html'
})
export class HomePage {
public params = {}
public pushPage = LocationPickerPage; // the page to be pushed
public pushTransPage = TransdetailPage;
public photosPage = SelectPhotosPage;
public sendLocation = SendLocationPage;
public selectContacts = SelectContactsPage;
constructor(public navCtrl: NavController) {
}
}
<file_sep>/src/pages/location-picker/location-picker.ts
import { Component, ChangeDetectorRef } from '@angular/core';
import { NavController, NavParams } from 'ionic-angular';
import {SelectContactsPage} from '../select-contacts/select-contacts'
/**
* Generated class for the LocationPickerPage page.
*
* See https://ionicframework.com/docs/components/#navigation for more info on
* Ionic pages and navigation.
*/
@Component({
selector: 'page-location-picker',
templateUrl: 'location-picker.html',
})
export class LocationPickerPage {
/** view vars used by ngui-maps */
autocomplete: any;
address: any = {};
center: any = "RR Nagar, Bengaluru, Karnataka, India";
code: string;
canContinue = false;
constructor(public navCtrl: NavController, public navParams: NavParams, private ref: ChangeDetectorRef) {
}
ionViewDidLoad() {
console.log('ionViewDidLoad LocationPickerPage');
}
// recenters the map to the location selected from auto complete
placeChanged(place) {
if(place && place.geometry){
this.center = place.geometry.location;
for (let i = 0; i < place.address_components.length; i++) {
let addressType = place.address_components[i].types[0];
this.address[addressType] = place.address_components[i].long_name;
}
this.address.name = place.name;
this.address.formatted_address = place.formatted_address;
this.canContinue = true;
}
else{
this.center = {};
this.address = {};
this.canContinue = false;
}
this.ref.detectChanges();
}
checkIfnotEmpty(event){
if(!event.target.value){
this.canContinue = false;
}
}
// push in the contacts select page
confirm(){
this.navCtrl.push(SelectContactsPage,this.address);
}
}
<file_sep>/src/pages/transdetail/transdetail-chat/chat-message/chat-message.ts
import { Component,Input } from '@angular/core';
/**
* Generated class for the ChatMessageComponent component.
*
* See https://angular.io/api/core/Component for more info on Angular
* Components.
*/
@Component({
selector: 'chat-message',
templateUrl: 'chat-message.html'
})
export class ChatMessageComponent {
text: string;
emojiOnly: string;
// TODO: get this value from service
currentUserID:string = '1';
@Input('data') data :any;
constructor() {
console.log('Hello ChatMessageComponent Component');
this.text = `It's a :laughing:`;
this.emojiOnly = `:laughing:`;
}
}
<file_sep>/src/components/avatar/avatar.ts
import { Component, Input } from '@angular/core';
/**
* Generated class for the AvatarComponent component.
*
* See https://angular.io/api/core/Component for more info on Angular
* Components.
*/
@Component({
selector: 'avatar',
templateUrl: 'avatar.html'
})
export class AvatarComponent {
initials: string;
@Input('img') img: any;
@Input('userName') set getInitials(val) {
try {
if (!val || val == "" || val == " ") {
this.initials = "?";
return;
};
let tmp = val.split(' ');
switch (tmp.length) {
case 1: {
this.initials = tmp[0].length > 1 ? tmp[0].split('')[0].toUpperCase() + tmp[0].split('')[1].toUpperCase() : tmp[0].split('')[0].toUpperCase();
break;
}
case 2: {
// make sure the second character is not a special character
this.initials = new RegExp(/[a-zA-Z]/).test(tmp[1].split('1')[0]) ? tmp[0].split('')[0].toUpperCase() + tmp[1].split('')[0].toUpperCase() : tmp[0].split('')[0].toUpperCase() + tmp[0].split('')[1].toUpperCase()
break;
}
case 0: {
this.initials = "?";
break;
}
default: {
// make sure the second character is not a special character
this.initials = new RegExp(/[a-zA-Z]/).test(tmp[1].split('1')[0]) ? tmp[0].split('')[0].toUpperCase() + tmp[1].split('')[0].toUpperCase() : tmp[0].split('')[0].toUpperCase() + tmp[0].split('')[1].toUpperCase()
break;
}
}
}
catch (e) {
this.initials = "?";
console.log('error with initials :', val)
}
}
constructor() {
console.log('Hello AvatarComponent Component');
}
}
<file_sep>/src/pages/transdetail/transdetail-chat/transdetail-chat.ts
import { Component,ViewChild , ElementRef} from '@angular/core';
import { NavController, NavParams } from 'ionic-angular';
import { Observable } from 'rxjs';
import {ChatServiceProvider} from "../../../providers/chat-service/chat-service"
/**
* Generated class for the TransdetailChatPage page.
*
* See https://ionicframework.com/docs/components/#navigation for more info on
* Ionic pages and navigation.
*/
@Component({
selector: 'page-transdetail-chat',
templateUrl: 'transdetail-chat.html',
providers: [ChatServiceProvider]
})
export class TransdetailChatPage {
chatName = "some random place";
private TransId: string ;
userDisplayName = "<NAME>";
currentUserID = 1; // this should come from a service or localstorage
message = '';
// actual messages shown in the window.
chatMessages:any = [];
private chatSubscription:any;
constructor(public navCtrl: NavController, public navParams: NavParams, private chatService: ChatServiceProvider) {
// get the trans id
this.TransId = this.navParams.data.transactionID;
// pre-populate the chat page with data received in navparams.
let chatHistory = navParams.data.chatHistory;
if(chatHistory && chatHistory.length){
chatHistory.map(chat=> this.onMessage(chat));
}
// start listening for new chats
this.chatSubscription = chatService.newMessage.subscribe(message=>{
this.onMessage(message);
});
}
ionViewDidLoad() {
console.log('ionViewDidLoad TransactionDetailsChatPage');
}
shouldAddStamp(message,index){
if(this.chatMessages.length >=2 && index!= 0 )
return !this.sameDay(message.time, this.chatMessages[index-1].time );
else
return true;
}
private sameDay(date1, date2) {
let d1 = new Date(date1);
let d2 = new Date(date2);
return d1.getFullYear() === d2.getFullYear() &&
d1.getMonth() === d2.getMonth() &&
d1.getDate() === d2.getDate();
}
// add the message in the chat window
onMessage(message) {
// create a new chat message object
let chatMessageObj ={
displayName: message.displayName,
userID: message.userID,
time: message.time,
data : [{
payload: message.data,
messageType : message.messageType
}]
}
console.log(chatMessageObj)
switch(this.chatMessages.length!=0){
case false: {
// push the existing chat message
this.chatMessages.push(chatMessageObj)
break;
}
case true: {
// if it's a message from the same user .. add it to the existing chat message obj
switch(chatMessageObj.userID === this.chatMessages[this.chatMessages.length-1].userID){
case true:{
// push the message into an existing chat message
this.chatMessages[this.chatMessages.length-1].data.push(chatMessageObj.data[0]);
break;
}
case false:{
this.chatMessages.push(chatMessageObj);
}
}
}
}
this.scrollToBottom()
}
sendMessage(message,type){
let chatMessageObj ={
displayName: this.userDisplayName,
userID: this.currentUserID,
messageType : new RegExp(/^@|:$/).test(message.trim()) == true ? 'emoji-only' : 'text' ,
data: message.trim(),
transactionID: this.TransId,
time:Date.now()
}
// send the message via the chat service :
this.chatService.send(chatMessageObj);
// clear the text area
this.message = '';
/**TODO: remove below code */
/** emulate other person talking */
let chatMessageObj2 ={
displayName: "<NAME>",
userID: 2,
messageType : new RegExp(/^@|:$/).test(message.trim()) == true ? 'emoji-only' : 'text' ,
data: message.trim(),
transactionID: this.TransId,
time:Date.now()
}
this.chatService.send(chatMessageObj2);
}
scrollToBottom(){
var element = document.getElementById("myScrollLabel");
setTimeout(()=>{element.scrollIntoView(true)},100);
}
getDateStamp(date){
let d = new Date(date);
return d;
}
}
| b62c5cfa05ab31b367bacbcaee47e1a0436314fe | [
"Markdown",
"TypeScript"
] | 13 | TypeScript | princenishchal/tllr | df5c42a5058f3001675fe45bc180d4724a940994 | 0af82966da331b7d9950e5e5c0965f60cfeb54fd | |
refs/heads/master | <repo_name>BI/react-step-wizard<file_sep>/src/components/slideReel.js
var React = require('react');
var PropTypes = require('prop-types');
var createReactClass = require('create-react-class');
require('../slideReelStyles.scss');
var SlideReel = createReactClass({
getInitialState: function() {
return {
};
},
propTypes: {
currentIndex: PropTypes.number.isRequired,
},
getSlides: function() {
return this.props.children.map(function (child, id) {
var style = {
left: id * 100 + "%",
};
return (
<div
key={id}
className="sr-slide"
style={style}>
{child}
</div>
)
}, this);
},
render: function() {
var slides = this.getSlides();
var style = {
left: -this.props.currentIndex * 100 + "%",
};
return (
<div className="sr-viewport">
<div className="sr-container">
<div className="sr-slidePanel" style={style}>
{slides}
</div>
</div>
</div>
);
}
});
module.exports = SlideReel;
<file_sep>/src/components/navigationButton.js
var React = require('react');
var PropTypes = require('prop-types');
var createReactClass = require('create-react-class');
var classNames = require('classnames');
var NavigationButton = createReactClass({
propTypes: {
stepData: PropTypes.object.isRequired,
onClick: PropTypes.func.isRequired,
isShown: PropTypes.bool.isRequired,
},
render: function () {
var stepData = this.props.stepData;
var description = null;
if(stepData.description !== null) {
description = (
<p className="sw-description">
{stepData.description}
</p>
);
}
var classes = classNames("sw-button", this.props.className, {"disabled": stepData.isUnreachable || !this.props.isShown});
return (
<button className={classes} onClick={this.props.onClick}>
<h1 className="sw-title">{stepData.title}</h1>
{description}
</button>
);
},
});
module.exports = NavigationButton;
<file_sep>/README.md
# react-step-wizard #
Step Wizard is comprised of two components: StepWizard (the main component) and Step (each step in the wizard). Step is a static property of StepWizard, and can be accessed with `StepWizard.Step`.
To view the demo, use the command `webpack` (install from npm--`npm install webpack -g`--if you don't have it) and open index.html.
## Development ##
* Development server `npm run dev`.
* Continuously run tests on file changes `npm run watch-test`;
* Run tests: `npm test`;
* Build `npm run build`;
## Properties ##
### StepWizard ###
(None yet)
### Step ###
* **title** (Required, string): The title that will be displayed on both the Step page and the navigation buttons.
* **description** (string, ""): If passed, it will be displayed on the both the Step page and the navigation buttons under the title.
* **onNext/onPrevious** (function(index), function(){}): Called when changing elements. If you change selected step using the pill selector up top, it will call each one in order.
* **onError** (function(index), function(){}): Called when you attempt to advance by clicking the navigation button but the current step is not valid.
* **isValid** (bool, true): Will update step wizard when changed. Does not allow progression beyond the first step with `isValid==false`, regardless of other steps' validity.
## Example Usage ##
var React = require('react');
var StepWizard = require('./src/stepWizard.js');
var Step = StepWizard.Step;
React.render(
<StepWizard>
<Step title="The First">
<p>This is the first step</p>
<p>This step is pretty cool, I guess</p>
</Step>
<Step title="The Second">
<p>This is the second step, it has an input tag</p>
<input type="text"/>
</Step>
<Step title="The Last" description="Whoa aren't descriptions neat?">
<p>This is the final step.</p>
<input type="button" value="Here's a button"/>
</Step>
</StepWizard>,
document.getElementById('content'));<file_sep>/src/components/navigationBeads.js
var React = require('react');
var PropTypes = require('prop-types');
var createReactClass = require('create-react-class');
var PillSelector = require('react-pill-selector');
var classNames = require('classnames');
var NavigationBeads = createReactClass({
getInitialState: function() {
return {};
},
propTypes: {
stepData: PropTypes.array.isRequired,
onClick: PropTypes.func.isRequired,
selectedIndex: PropTypes.number.isRequired,
},
makeBead: function(step, index) {
var classes = classNames("sw-bead", {"sw-bead-unreachable": step.isUnreachable});
var isDisabled = false;//step.isUnreachable;
return (
<li key={index} className={classes} isDisabled={isDisabled}>
{step.title}
</li>
);
},
onItemClick: function(id) {
this.props.onClick(id);
},
render: function () {
var beads = this.props.stepData.map(this.makeBead, this);
return (
<PillSelector
callClickOnLoad={false}
onItemClicked={this.onItemClick}
selectedIndex={this.props.selectedIndex}
isManaged={true}>
{beads}
</PillSelector>
);
},
});
module.exports = NavigationBeads;
| ca5d0d503b7ce7bea17d8b5398b99998562d1dde | [
"JavaScript",
"Markdown"
] | 4 | JavaScript | BI/react-step-wizard | ee5fc6ac6d813990ac751d0065c245d3b2a95c1e | f1340729fd43010fc026f3741d5b39bec407946e | |
refs/heads/master | <repo_name>PSGXerus/volumio-plugins<file_sep>/plugins/miscellanea/ambx/index.js
'use strict';
var libQ = require('kew');
var fs=require('fs-extra');
var config = new (require('v-conf'))();
var exec = require('child_process').exec;
var execSync = require('child_process').execSync;
module.exports = ambx;
function ambx(context) {
var self = this;
this.context = context;
this.commandRouter = this.context.coreCommand;
this.logger = this.context.logger;
this.configManager = this.context.configManager;
}
ambx.prototype.onVolumioStart = function()
{
var self = this;
var configFile=this.commandRouter.pluginManager.getConfigurationFile(this.context,'config.json');
this.config = new (require('v-conf'))();
this.config.loadFile(configFile);
return libQ.resolve();
}
ambx.prototype.onStart = function() {
var self = this;
var defer=libQ.defer();
var color = self.config.get('colorHex');
if (typeof color === 'undefined') {
color = "#000000";
}
color = color.match(/[A-Za-z0-9]{2}/g).map(function(v) { return parseInt(v, 16) });
exec("ambx_set_color -r " + color[0] + " -g " + color[1] + " -b " + color[2], function (error, stdout, stderr) {
if(error){
self.logger.info('No control on AmbX');
defer.reject(new Error());
} else {
// Once the Plugin has successfull started resolve the promise
defer.resolve();
}
});
return defer.promise;
};
ambx.prototype.onStop = function() {
var self = this;
var defer=libQ.defer();
exec("ambx_set_color -o", function (error, stdout, stderr) {
if(error){
self.logger.info('No control on AmbX');
defer.reject(new Error());
} else {
// Once the Plugin has successfull stopped resolve the promise
defer.resolve();
}
});
return defer.promise;
};
ambx.prototype.onRestart = function() {
var self = this;
// Optional, use if you need it
};
// Configuration Methods -----------------------------------------------------------------------------
ambx.prototype.getUIConfig = function() {
var defer = libQ.defer();
var self = this;
var lang_code = this.commandRouter.sharedVars.get('language_code');
self.commandRouter.i18nJson(__dirname+'/i18n/strings_'+lang_code+'.json',
__dirname+'/i18n/strings_en.json',
__dirname + '/UIConfig.json')
.then(function(uiconf)
{
uiconf.sections[0].content[0].value = self.config.get('colorHex');
defer.resolve(uiconf);
})
.fail(function()
{
defer.reject(new Error());
});
return defer.promise;
};
ambx.prototype.setColor = function(data) {
var self = this;
var defer = libQ.defer();
var color = data['colorHex']
self.config.set('colorHex', color);
color = color.match(/[A-Za-z0-9]{2}/g).map(function(v) { return parseInt(v, 16) });
exec("ambx_set_color -r " + color[0] + " -g " + color[1] + " -b " + color[2], function (error, stdout, stderr) {
if(error){
self.commandRouter.pushToastMessage('error', "Set Color", "No connection to AmbX");
defer.reject(new Error());
} else {
self.commandRouter.pushToastMessage('success', "Set Color", "Set color was successful");
defer.resolve({});
}
});
return defer.promise;
}
ambx.prototype.getConfigurationFiles = function() {
return ['config.json'];
}
ambx.prototype.setUIConfig = function(data) {
var self = this;
//Perform your installation tasks here
};
ambx.prototype.getConf = function(varName) {
var self = this;
//Perform your installation tasks here
};
ambx.prototype.setConf = function(varName, varValue) {
var self = this;
//Perform your installation tasks here
};
<file_sep>/plugins/miscellanea/ambx/uninstall.sh
#!/bin/bash
# Uninstall dependendencies
sudo rm /etc/udev/rules.d/10-ambx.rules
sudo rm -r /usr/lib/python2.7/dist-packages/ambx
echo "Done"
echo "pluginuninstallend"
<file_sep>/plugins/miscellanea/ambx/ambx_set_color
#!/usr/bin/env python
# -*- coding: utf-8 -*-
from ambx.ambx import AMBX, Lights
import struct
import sys, getopt
lights = [Lights.LEFT, Lights.RIGHT, Lights.WWLEFT, Lights.WWCENTER, Lights.WWRIGHT]
def print_help():
print 'ambx_set_color [ -h | -o | -r <red> -g <green> -b <blue>]'
def set_color(red, green, blue):
dev = None
try:
dev = AMBX(0)
for light in lights:
try:
dev.set_color_rgb8(light, [red, green, blue])
except IOError:
print 'USB Error'
break
except IndexError:
if dev is None:
print 'No AmbX found!'
if __name__ == '__main__':
argv = sys.argv[1:]
try:
opts, args = getopt.getopt(argv,"hor:g:b:",["help", "red=","green=","blue=", "off"])
except getopt.GetoptError:
print_help()
sys.exit(2)
for opt, arg in opts:
if opt in ("-h", "--help"):
print_help()
sys.exit()
elif opt in ("-r", "--red"):
red = int(arg)
elif opt in ("-g", "--green"):
green = int(arg)
elif opt in ("-b", "--blue"):
blue = int(arg)
elif opt in ("-o", "--off"):
red = 0
green = 0
blue = 0
set_color(red, green, blue)
<file_sep>/plugins/miscellanea/ambx/install.sh
#!/bin/bash
echo "Installing PyUSB"
sudo apt update
sudo apt -y install python-pip --no-install-recommends
sudo pip install pyusb
echo "Installing color change executable"
sudo sh -c 'curl -L https://raw.githubusercontent.com/PSGXerus/ambx-sound-light/master/10-ambx.rules > /etc/udev/rules.d/10-ambx.rules'
sudo cp ambx_set_color /usr/bin
sudo mkdir -p /usr/lib/python2.7/dist-packages/ambx
sudo touch /usr/lib/python2.7/dist-packages/ambx/__init__.py
sudo sh -c 'curl -L https://raw.githubusercontent.com/PSGXerus/ambx-sound-light/master/ambx/ambx.py > /usr/lib/python2.7/dist-packages/ambx/ambx.py'
echo "plugininstallend"
| ce4b99c30645f253d34982cf1d1b4929842b5de9 | [
"JavaScript",
"Python",
"Shell"
] | 4 | JavaScript | PSGXerus/volumio-plugins | 7fb38b07878768f3dab4fae7e509ffeb0e2b661a | fe52929957158187458127a1685989caf578a398 | |
refs/heads/master | <file_sep>function swap(arr, index) {
var oldLeft = arr[index];
arr[index] = arr[index + 1];
arr[index + 1] = oldLeft;
return arr;
}
function bubbleSort(array) {
var count = 1;
while (count) {
count = 0;
for (var i = 0; i < array.length - 1; i++) {
if (array[i] > array [i+1]) {
array = swap(array, i);
count++;
}
}
}
return array;
}
<file_sep>//--------- Bubble Sort
//empty array
describe('Bubble Sort', function(){
it('handles an empty array', function(){
expect( bubbleSort([]) ).toEqual( [] );
});
});
//single items
describe('Bubble Sort', function(){
it('handles an array of length 1', function(){
expect( bubbleSort([5]) ).toEqual( [5] );
});
});
// multiple items
describe('Bubble Sort', function(){
it('handles an array with multiple elements', function(){
expect( bubbleSort([2, 3, 1]) ).toEqual( [1, 2, 3] );
});
});
describe('Bubble Sort', function(){
it('handles an array with multiple elements', function(){
expect( bubbleSort([1, 3, 1, 5, 7]) ).toEqual( [1, 1, 3, 5, 7] );
});
});
<file_sep># sorting
Implementation of bubble and merge sorting algorithms
<file_sep>describe('Split Array function', function() {
it('is able to split an array into two halves', function() {
expect(split([1,2,3,4])).toEqual([[1,2],[3,4]]);
});
});
describe('Split Array function', function() {
it('is able to split an odd array into two halves', function() {
expect(split([1,2,3,4,5])).toEqual([[1,2],[3,4,5]]);
});
});
describe('Merge Sort', function(){
it('is able to merge two sorted arrays into one sorted array', function(){
expect(merge([1],[2])).toEqual([1,2]);
});
});
describe('Merge Sort', function(){
it('is able to merge two sorted arrays into one sorted array', function(){
expect(merge([1,3], [2,4])).toEqual([1,2,3,4]);
});
});
describe('Mergesort', function(){
it('fdsfdsfsdfsd', function(){
expect(mergeSort([4,1,5,9,3,8])).toEqual([1,3,4,5,8,9])
});
});
describe('Mergesort', function(){
it('fdsfdsfsdfsd', function(){
expect(mergeSort([1,4,5,9,3,1,8])).toEqual([1,1,3,4,5,8,9])
});
});
<file_sep>function split(wholeArray) {
var firstHalf = wholeArray.slice(0, wholeArray.length / 2);
var secondHalf = wholeArray.slice(wholeArray.length / 2);
return [firstHalf, secondHalf];
}
function merge(left, right){
var output = [],
leftIndex = 0,
rightIndex = 0;
while (leftIndex < left.length && rightIndex < right.length) {
if (left[leftIndex] < right[rightIndex]) {
output.push(left[leftIndex]);
leftIndex++;
}
else {
output.push(right[rightIndex]);
rightIndex++;
}
}
for (; leftIndex < left.length; leftIndex++) output.push(left[leftIndex]);
for (; rightIndex < right.length; rightIndex++) output.push(right[rightIndex]);
return output;
}
function mergeSort(array) {
if (array.length > 1) {
var arrLeft = split(array)[0];
var arrRight = split(array)[1];
return merge(mergeSort(arrLeft), mergeSort(arrRight));
}
return array;
}
| 953c5923500f0a8072af79dc59a7190703d7f0f6 | [
"JavaScript",
"Markdown"
] | 5 | JavaScript | avillr/sorting | 3d9e8e7b416a078fa89312765b2df2c01d3672de | 2ab880e552681c4c04fe737cc94a0438fa059bd2 | |
refs/heads/master | <repo_name>davenporta/live_graphs<file_sep>/README.md
# live_graphs
Live graph dashboard for MASA testing
-----------------------
### Plot Configuration
Easily configurable with graph_settings.csv file
Each row is a separate plots
Add as many as you'd like
Column | Function
------ | --------
type | not yet implemented
title | title of plot
x | pandas dataframe header for x axis data
xlabel | x axis label
y | y data set header in database (divide with " \| ")
alias | data title in legend (divide with " \| ")
pen | curve style field (divide with " \| ")
ylabel | y axis label
row, col | subplot layout position
seconds | seconds of data to plot (up to `seconds_to_store`)
### Required Python Libraries
* PyQt5
* PyQtGraph
* Pandas
* NumPy
### Task List
- [ ] proper data stream to dataframe (networking)
- [ ] add numerical fields (not just graphs)
- [ ] mathematical operations on datasets (for calculating total thrust or pressure drop or what not)
- [ ] figure out window sizing
- [ ] pretty things up
- [ ] settings menu
<file_sep>/live_graph.py
import pandas as pd
from PyQt5 import QtGui
from PyQt5.QtCore import Qt
import pyqtgraph as pg
import time
import numpy as np
import pandas as pd
from PlotDefinition import PlotDefinition
#TODO: proper data stream (database?)
#TODO: add numerical fields (not just graphs)
#TODO: mathematical operations on datasets (for calculating total thrust or pressure drop or what not)
#TODO: figure out window sizing (WTF WHY U NO WORK NO MATTER WHAT I TRY THE COLUMNS ARE DIFFERENT SIZES)
#window title
run_name = "MASA Live Data Dashboard"
#initialize Qt
app = QtGui.QApplication([])
#timer
start_time = pg.ptime.time()
#top level
top = QtGui.QMainWindow()
w = QtGui.QWidget()
top.setCentralWidget(w)
top.setWindowTitle(run_name)
app.setWindowIcon(QtGui.QIcon('logos/logo.png'))
# layout grid
layout = QtGui.QGridLayout()
w.setLayout(layout)
# Zero Indexes for the gui layout (row, column) (sternie's idea)
zr = 0
zc = 0
#load app settings (in the future will enable a settings menu)
app_settings = pd.read_csv("app_settings.csv")
#load graph settings
graph_settings = pd.read_csv(app_settings['graph_settings_path'][0])
#number of points to store given tick_rate and seconds_to_store
def tickCalc(tr, s):
return int(s/(tr/1000))
#max number of datapoints to store/retrieve
tick_rate = app_settings['tick_rate'][0] #in ms (calculated limit at about 35-40 ms)
seconds_to_store = graph_settings['seconds'].max() #save as much memory as possible (keep only what's needed)
data_range = tickCalc(tick_rate, seconds_to_store) #this isn't right and I don't know why
#last_time = pg.ptime.time()
#initialize data arrays (for testing only)
#eventually load data from web database into dataframe
cols = ['time', 'cos', 'neg_cos', 'destruc']
database = pd.DataFrame(columns=cols)
#add area for tiled plots
plot_box = pg.GraphicsLayoutWidget()
layout.addWidget(plot_box, zr+0, zc+0)
#status = QtGui.statusBar(top)
mainMenu = top.menuBar()
mainMenu.setNativeMenuBar(True)
fileMenu = mainMenu.addMenu('&File')
dataMenu = mainMenu.addMenu('&Data')
#quit application function
#in case more shutdown actions are needed later
def exit():
app.quit()
#quit application menu item
quit = QtGui.QAction("&Quit", fileMenu)
quit.setShortcut("Ctrl+Q")
quit.triggered.connect(exit)
fileMenu.addAction(quit)
#clear data function
def clear():
database.drop(database.index, inplace=True)
#clear data menu item
clear_data = QtGui.QAction("&Clear Data", dataMenu)
clear_data.setShortcut("Ctrl+D")
clear_data.triggered.connect(clear)
dataMenu.addAction(clear_data)
#list for easy iteration through plots
plots = []
#for each plot in parameters
for i in range(len(graph_settings.index)):
#get parameters for plot
row = graph_settings.ix[i]
#plot location on screen
subplot = (row['row'], row['col'])
#initialize plot and add to list
plots.append(PlotDefinition(subplot, title=row['title'], xlabel=row['xlabel'], ylabel=row['ylabel'], data_range=tickCalc(tick_rate, row['seconds'])))
#set x data
plots[i].setX(row['x'])
#parse y data
ys = row['y'].split(' | ')
pens = row['pen'].split(' | ')
aliases = row['alias'].split(' | ')
params = zip(ys, pens, aliases)
#push y data to plot
for param in params:
plots[i].addY(param)
#make plot and push to window
plots[i].makePlot(plot_box, show_legend=bool(row['legend']), show_grid=bool(row['grid']))
#fake data stream in place of serial or some other type of read in
def fake_data():
t = pg.ptime.time() - start_time
y1 = np.cos(2 * np.pi * (t%500))
y2 = -y1
y3 = y1+y2
return [t,y1,y2,y3]
#update function runs on each tick
def update():
global database, cols, last_time
#print(str((pg.ptime.time()-last_time)*1000-tick_rate) + " ms lag time this tick")
#last_time = pg.ptime.time()
#get data
t,y1,y2,y3 = fake_data()
#update database
database = database.append(pd.DataFrame([[t,y1,y2,y3]],columns=cols))
#slice off out of range data
database = database.tail(data_range)
#update plots with new data
for p in plots:
p.updatePlot(database)
#display window
#using .showMaximized() instead of .show() until I can figure out sizing
top.showMaximized()
#timer and tick updates
timer = pg.QtCore.QTimer()
timer.timeout.connect(update)
timer.start(tick_rate)
#start application (Qt Loop Cycle)
app.exec_()
| 4b1e54887b072ad965e1cb980f385e8634df955d | [
"Markdown",
"Python"
] | 2 | Markdown | davenporta/live_graphs | b192f5273976b18a0c2fdefa0faf0b0c1ef67b07 | 7c64d3c3e6508f972426eda0816dca3833c068f3 | |
refs/heads/master | <repo_name>vyasnikul/GoPrac<file_sep>/examples/Hello/main.go
package main
import (
"fmt"
)
func linearSearch(arr []int, key int) bool {
for i, val := range arr {
if val == key {
fmt.Println(i, ":", val)
return true
}
}
return false
}
func binarySearch(arr []int, key int) bool {
min := 0
max := len(arr) - 1
median := 0
for min <= max {
median = (min + max) / 2
if arr[median] < key {
min = median + 1
} else {
max = median - 1
}
}
if min == len(arr) || arr[min] != key {
return false
}
fmt.Println(median, ":", key)
return true
}
func interpolationSearch(arr []int, key int) int {
Length := len(arr)
min, max := arr[0], arr[Length-1]
low, high := 0, Length-1
for {
if key < min {
return low
}
if key > max {
return Length
}
// Making guess of location
guess := 0
if high == low {
guess = high
} else {
size := high - low
offset := int(float64(size-1) * (float64(key-min) / float64(max-min)))
guess = low + offset
}
// If element is found
if arr[guess] == key {
fmt.Println(guess, ":", key)
return guess
}
// if guess is high, go lower direction or vice versa
if arr[guess] > key {
high = guess - 1
max = arr[high]
} else {
low = guess + 1
min = arr[low]
}
}
}
func quickSort(arr []int) []int {
Length := len(arr)
if Length < 2 {
return arr
}
low, high := 0, Length-1
pivot := int((Length - 1) / 2)
arr[pivot], arr[high] = arr[high], arr[pivot]
for index := range arr {
if arr[index] < arr[high] {
arr[low], arr[index] = arr[index], arr[low]
low++
}
}
arr[low], arr[high] = arr[high], arr[low]
quickSort(arr[:low])
quickSort(arr[low+1:])
return arr
}
func main() {
xs := []int{95, 78, 46, 58, 45, 86, 99, 251, 320}
fmt.Println("<!DOCTYPE html>")
fmt.Println("<html>")
fmt.Println("<head>My First Web Page in Go</head>")
fmt.Println("<body>")
fmt.Println("Input Array: ", xs)
fmt.Println("Linear Search Output:", linearSearch(xs, 99))
fmt.Println("Binary Search Output:", binarySearch(xs, 86))
fmt.Println("Interpolation Search Output:", interpolationSearch(xs, 251))
fmt.Println("Quick Sort : ", quickSort(xs))
fmt.Println("</body>")
fmt.Println("</html>")
}
<file_sep>/examples/DS/hw1.go
package main
import "fmt"
// PrintEvenNumbers : Printing Even numbers using for loop
func PrintEvenNumbers(x int) {
// Using For
for i := 0; i <= x; i++ {
if i%2 == 0 {
fmt.Println(i)
}
}
}
// CheckPrime : Check the number is prime or not.
func CheckPrime(x int) {
count := 0
for i := 1; i < x/2; i++ {
if x%i == 0 {
count++
}
}
if count == 1 {
fmt.Println(x, " is prime.")
} else {
fmt.Println(x, " is not prime.")
}
}
// findKIndex : find index of elements leading to value k
func findKIndex(arr []int, k int) (int, int) {
index1, index2 := 0, 0
for idx1, val1 := range arr {
for idx2, val2 := range arr[idx1+1:] {
if val1+val2 == k {
index1 = idx1
index2 = idx1 + idx2 + 1
}
}
}
return index1, index2
}
func main() {
x := 0
xs := []int{2, 4, 5, 6, 7, 8, 10, 10}
fmt.Println("Enter the limit for Even numbers:")
fmt.Scanf("%d", &x)
fmt.Println("===========RESULT===============")
PrintEvenNumbers(x)
fmt.Println()
CheckPrime(x)
fmt.Println()
fmt.Println(findKIndex(xs, x))
}
| 56cf91e712d6299e8802af4cc963819ed38c3283 | [
"Go"
] | 2 | Go | vyasnikul/GoPrac | 4dabaa8fc5cf361550021b42859b8ba3ac647664 | e1680d9ee79d94821fab9b1b63ed3c95246e9601 | |
refs/heads/master | <file_sep>$:.unshift File.expand_path('../lib', __FILE__)
require 'eventmachine'
require 'json'
require 'ayaneru'
run Ayaneru::Server
EM::defer do
yesterday = Time.now.day - 1
loop do
today = Time.now.day
if today != yesterday
registered_tags = Ayaneru.redis.lrange 'tags', 0, -1
registered_tags.each do |tag|
r = Ayaneru.niconico.search(tag, 1).to_s.split("\n")
results = JSON.parse(r[2])
if results['values']
results['values'].each do |value|
begin
ret = Ayaneru.niconico.reserve(value["cmsid"])
rescue => exception
puts exception.message
begin
Ayaneru.twitter.create_direct_message(Ayaneru.twitter.user.screen_name, "これ以上タイムシフト予約できません.『#{value['title']}』(http://live.nicovideo.jp/watch/#{value['cmsid']})")
rescue => exception
puts exception.message
end
end
begin
Ayaneru.twitter.create_direct_message(Ayaneru.twitter.user.screen_name, "『#{value['title']}』(http://live.nicovideo.jp/watch/#{value['cmsid']})をタイムシフト予約しました.") if ret
rescue => exception
puts exception.message
end
end
end
end
yesterday = today
end
sleep 60*60*1
end
end
<file_sep>require 'json'
module Ayaneru
class Niconico
def search(tag, until_days)
logout
post_data = {}
data_filters = Array.new
data_filters[0] = {
"field" => "ss_adult",
"type" => "equal",
"value" => false
}
data_filters[1] = {
"field" => "live_status",
"type" => "equal",
"value" => "reserved"
}
data_filters[2] = {
"field" => "provider_type",
"type" => "equal",
"value" => "official"
}
data_filters[3] = {
"field" => "start_time",
"from" => Time.now.strftime("%Y-%m-%d %H:%M:%S"),
"include_lower" => true,
"include_upper" => true,
"to" => Time.at(Time.now.to_i + until_days * 24 * 60 * 60).strftime("%Y-%m-%d %H:%M:%S"),
"type" => "range"
}
data_filters[4] = {
"field" => "timeshift_enabled",
"type" => "equal",
"value" => true
}
post_data["filters"] = data_filters
post_data["from"] = 0
post_data["issuer"] = "http://github.com/tsuwatch/autots"
post_data["join"] = [
"cmsid",
"title",
"description",
"community_id",
"open_time",
"start_time",
"live_end_time",
"comment_counter",
"score_timeshift_reserved",
"provider_type",
"channel_id",
"live_status",
"tags",
"member_only"
]
post_data["order"] = "desc"
post_data["query"] = tag
post_data["reason"] = "default"
post_data["search"] = ["tags"]
post_data["service"] = ["live"]
post_data["size"] = 100
post_data["sort_by"] = "_live_recent"
post_data["timeout"] = 10000
response = Ayaneru.niconico.agent.post(URL[:search], JSON.pretty_generate(post_data))
response.body
end
end
end
<file_sep>FROM ruby:2.2.3
WORKDIR /app
ADD Gemfile /app/Gemfile
ADD Gemfile.lock /app/Gemfile.lock
RUN bundle install -j4
ADD . /app
<file_sep># A sample Gemfile
source "https://rubygems.org"
gem "sinatra"
gem "haml"
gem "mechanize"
gem "redis"
gem 'twitter'
gem 'eventmachine'
gem 'thin'
gem 'dotenv'
<file_sep>require 'sinatra/base'
require 'haml'
module Ayaneru
class Server < Sinatra::Base
use Rack::Auth::Basic, "Restricted Area" do |username, password|
username == ENV['BASIC_USERNAME'] and password == ENV['BASIC_<PASSWORD>']
end
set :bind, '0.0.0.0'
get '/' do
@registered_tags = Ayaneru.redis.lrange 'tags', 0, -1
@results = {}
@registered_tags.each do |tag|
chunks = Ayaneru.niconico.search(tag, 7).to_s.split("\n")
chunks.each do |chunk|
row = JSON.parse(chunk)
next if !(row.has_value?('hits') and row.key?('values'))
@results[tag] = row
break
end
end
haml :index
end
post '/' do
Ayaneru.redis.rpush "tags", params[:tag]
redirect '/'
end
post '/delete' do
Ayaneru.redis.lrem "tags", 1, params[:tag]
redirect '/'
end
end
end
| 640508eb4b4c5f972634e1a79655c2ede538f556 | [
"Ruby",
"Dockerfile"
] | 5 | Ruby | Miutaku/autots | c3dcf0527255087ea0e5b050eef51ad8cb3ffc04 | f47c887d5cc51d755463caddef9eb3d5c78b50cd | |
refs/heads/master | <repo_name>bugra/karavela<file_sep>/upgrade.sh
pip install caravel --upgrade
caravel db upgrade
<file_sep>/ubuntu.sh
sudo apt-get install build-essential libssl-dev libffi-dev python-dev python-pip
sudo pip install numpy
sudo pip install caravel
<file_sep>/docker.sh
docker rm -f caravel
docker run --detach --name caravel \
--env SECRET_KEY=$CARAVEL_SUPERSECRET_KEY \
--env SQLALCHEMY_DATABASE_URI=$CARAVEL_URL\
--publish 8088:8088 \
amancevice/caravel
docker exec caravel caravel db upgrade
# Create default roles and permissions
docker exec caravel caravel init
| 312a503794e5cf789a7783c91bd19e3ae011eb3c | [
"Shell"
] | 3 | Shell | bugra/karavela | ee8682fe0311424843dbd5c7cb59fab5c47a033f | e5fee57d3ddc88e2c727d590134b423b08682988 | |
refs/heads/master | <file_sep>function basicTeenager(age) {if (age>=13&&age<=19) {return 'You are a teenager!';}
}
function teenager(age) {if (age>=13&&age<=19) {return 'You are a teenager!';} else {return 'You are not a teenager';}
}
function ageChecker(age) {if (age>=13&&age<=19) {return 'You are a teenager!';} else if(age<=12) {return 'You are a kid'}
else if(age>=20) {return 'You are a grownup';}
}
function ternaryTeenager(age) { if (age>=13&&age<=19) {return 'You are a teenager';} else {return 'You are not a teenager';}
}
function switchAge(age) { if (age>=13&&age<=19) {return 'You are a teenager';} else {return 'You have an age';}
}
| 32e2e819cc4a577d4009116a0f948d4a3af9d103 | [
"JavaScript"
] | 1 | JavaScript | NicolaasJ/skills-based-javascript-intro-to-flow-control-js-intro-000 | ec56af0908d5ea5cc0b68062c3b01508df1c9068 | 8fc5cbd46900c12bf4a05371f57df1723e4920c7 | |
refs/heads/master | <file_sep>plotRoute <- function(graph, nodes, ...) {
lines(V(graph)[nodes]$x, V(graph)[nodes]$y, ...)
}
<file_sep>makeNest <- function(n) {
### generate a bunch of SpatialLines on (0,1) square
xy = cbind(runif(n), runif(n), runif(n), runif(n))
lines = Lines(apply(xy, 1, function(z) {
Line(rbind(z[1:2], z[3:4]))
}), ID = 1)
SpatialLines(list(lines))
}
<file_sep>routePoints <- function(graph, from, to) {
require(FNN)
xyg = cbind(V(graph)$x, V(graph)$y)
ifrom = get.knnx(xyg, from, 1)$nn.index[1, 1]
ito = get.knnx(xyg, to, 1)$nn.index[1, 1]
p = get.shortest.paths(graph, ifrom, ito, output = "vpath")
p[[1]]
}
<file_sep>
Running Up That Hill
======================
<NAME>
-------------------
After figuring out how to make `igraph` network structures from lines I turned my thoughts to creating flow networks from river lines.
Often you'll get a shapefile of river data with neither topology nor flow direction. This prevents you answering questions such as:
* A river is polluted at some given point. Identify all the downstream polluted areas.
* Pollution is detected at some point. Identify all the upstream tributaries that the pollution may have come from.
By overlaying the river network on a digital elevation model (DEM) it should be possible to work out which way river segments flow.
```{r echo=FALSE}
set.seed(310366)
options(warn=-1)
opts_chunk$set(tidy=TRUE,cache=TRUE)
riverDataDir = "/home/rowlings/Work/R/UseR/UseR2012/Tutorial/Data"
lakeDataDir = "/home/rowlings/Work/R/UseR/UseR2012/Tutorial/Data/"
demDataDir = "/home/rowlings/Downloads"
```
Requirements
--------------
We need some packages
```{r warning=FALSE,message=FALSE,results="hide"}
require(igraph)
require(sp)
require(rgdal)
require(raster)
require(rgeos)
```
Data
------
My map data is stored in a few different directories. The river and lake data comes from GeoFabrik's Cumbria shapefile, which is derived from OpenStreetMap sources. The digital elevation model is from NASA's SRTM project. All this data is freely available.
```{r readData}
rivers = readOGR(riverDataDir,"rivers")
lakes = readOGR(lakeDataDir,"naturalwater")
dem = raster(file.path(demDataDir,"cumbriaDem.tif"))
```
We need to work in a single projection, so we transform the vector data to the projection of the raster. This is a Lambert Equal Area projection in metres.
```{r projections}
projection(dem)
lakesP = spTransform(lakes,CRS(projection(dem)))
riversP = spTransform(rivers,CRS(projection(dem)))
```
Joining Lakes and Rivers
--------------------------
When plotting the rivers you will notice big gaps where the lakes are. This is going to interrupt our graph network. One way round this is to incorporate the lake edges into the network by adding them to the rivers to create one larger `SpatialLines` object. Any routing through the lake will choose a path round the edge of the lake. Ideally you might want to create a single network vertex for the lake and join the incoming and outgoing streams to this.
There are a lot of small lakes - mostly ponds and mountain tarns - in the lakes shapefile, and these don't affect teh river network. A bit of trial and error helps us find an area threshold that takes out these features. We then plot our new water data - a `SpatialLines` object - on top of the digital elevation model.
```{r lakethin}
joinLR <- function(lakes,rivers,lakesize){
area = gArea(lakes,byid=TRUE)
lakes = lakes[area>lakesize,]
lakelines = as(lakes,"SpatialLines")
water = gUnion(lakelines,rivers)
return(water)
}
water = joinLR(lakesP,riversP,2e5)
plot(dem)
lines(water)
```
Making The Network
--------------------
We will use the same functions as before to build the topology and connectivity of the network.
```{r buildtopo}
buildTopo = function(lines) {
g = gIntersection(lines, lines)
edges = do.call(rbind, lapply(g@lines[[1]]@Lines, function(ls) {
as.vector(t(ls@coords))
}))
lengths = sqrt((edges[, 1] - edges[, 3])^2 + (edges[, 2] - edges[, 4])^2)
froms = paste(edges[, 1], edges[, 2])
tos = paste(edges[, 3], edges[, 4])
graph = graph.edgelist(cbind(froms, tos), directed = FALSE)
E(graph)$weight = lengths
xy = do.call(rbind, strsplit(V(graph)$name, " "))
V(graph)$x = as.numeric(xy[, 1])
V(graph)$y = as.numeric(xy[, 2])
return(graph)
}
plotRoute <-
function(graph, nodes, add=FALSE,...) {
if(add){
lines(V(graph)[nodes]$x, V(graph)[nodes]$y, ...)
} else {
plot(V(graph)[nodes]$x, V(graph)[nodes]$y, type="l", ...)
}
}
g = buildTopo(water)
```
Extracting The Heights
------------------------
Assigning the elevation of the DEM to each node is simple a matter of using the `extract` function of the `raster` package.
```{r heights}
xy = cbind(V(g)$x,V(g)$y)
z=extract(dem,xy)
V(g)$z=z
```
We now have a three-dimensional network of rivers.
River Height Profile
----------------------
We can investigate how the altitude of a river changes as it flows downstream.
As long as the river network is a tree then if we choose an upland start point and the river mouth as end point we should find that the shortest route is also the downhill route of the river. We'll choose two points to follow Langstrath Beck as it passes through two lakes to become the Derwent River which meets the sea at Whitehaven.
```{r langstrath}
from = cbind(3475014, 3562463)
to = cbind( 3452108, 3586383)
pp = routePoints(g,from,to)
plotRoute(g,pp,col="blue",lwd=2,asp=1,xlab="",ylab="")
plot(water,add=TRUE)
```
To plot the altitude against distance we simply compute the cumulative pythagorean distance of the nodes of the route against the altitude obtained from sampling the DEM.
```{r elevationPlot}
dpath = c(0,cumsum(sqrt(diff(V(g)[pp]$x)^2 + diff(V(g)[pp]$y)^2)))
plot(dpath/1000,V(g)[pp]$z,type="l",xlab="distance/m",ylab="height above sea level/km")
```
Oh dear. This river clearly flows uphill. Sometimes as much as 25 metres. Rivers don't do this, so it must be errors in the DEM or the river line.
One thing that can be seen is the overall change in behaviour of the river. At the start the small stream tumbles steeply down the mountains, then reaches a middle region where it flows into the lakes. These lakes can be seen as two flat-bottomed parts of the profile at about 12km and 25km. After leaving the second lake, and now a river, it rolls gently down to the sea.
Deriving a Flow Network
-------------------------
Can we derive a flow network from this data? Obviously this plot shows that line segments seem to go up as well as down. We might get something more usable by smoothing the DEM or the sampled heights. Another possibility is to not sample at every point, but to ignore nodes with two edges, since any flow along a series of nodes with two edges has to be in the same direction. By only testing the heights at the nodes that occur at confluences, we will be sampling over a larger drop and hopefully the noise in the DEM won't be enough to affect the resulting direction. This will probably be the subject of the next chapter.
<file_sep>buildTopo <- function(lines) {
g = gIntersection(lines, lines)
edges = do.call(rbind, lapply(g@lines[[1]]@Lines, function(ls) {
as.vector(t(ls@coords))
}))
lengths = sqrt((edges[, 1] - edges[, 3])^2 + (edges[, 2] - edges[, 4])^2)
froms = paste(edges[, 1], edges[, 2])
tos = paste(edges[, 3], edges[, 4])
graph = graph.edgelist(cbind(froms, tos), directed = FALSE)
E(graph)$weight = lengths
xy = do.call(rbind, strsplit(V(graph)$name, " "))
V(graph)$x = as.numeric(xy[, 1])
V(graph)$y = as.numeric(xy[, 2])
return(graph)
}
<file_sep>joinLR <- function(lakes,rivers,lakesize){
area = gArea(lakes,byid=TRUE)
lakes = lakes[area>lakesize,]
lakelines = as(lakes,"SpatialLines")
water = gUnion(lakelines,rivers)
return(water)
}
makeG <- function(water){
return(buildTopo(water))
}
<file_sep>geoutils
========
Assorted map utilities<file_sep># get all lakes >=2e5 m^2
water = joinLR(lakesP,riversP,2e5)
g = makeG(water)
plot(dem2)
lines(water)
xy = cbind(V(g)$x,V(g)$y)
z=extract(dem2,xy)
V(g)$z=z
<file_sep>smoother <- function(g){
### smooth graph by removing degree=2 vertices
###
## label the vertices
g = set.vertex.attribute(g,name="ID",value=paste("V",1:length(V(g)),sep=""))
## find all degree-2 vertices
twos = V(g)[degree(g)==2]
## subgraph with only those
subG = induced.subgraph(g,twos)
## now we're going to delete all these nodes.
## but we're going to add edges between the bits on the end of each string-of-pearls
strings = clusters(subG)
for(i in 1:strings$no){
string = V(subG)[strings$membership == i]
ends = V(subG)[strings$membership == i & degree(subG)==1]
if(length(ends)!=2){
warning("short cluster")
next
}
endNeighs = c(neighbors(g,V(g)[ends$name[1]]),neighbors(g,V(g)[ends$name[2]]))
endDegree = degree(g,endNeighs)
endPoints = V(g)[endNeighs[endDegree!=2]]$name
# now we have the new edge points
g = add.edges(g,endPoints)
g = delete.vertices(g,string$name)
}
g
}
<file_sep>pathlength <- function(pts){
dx = diff(pts$x)^2
dy = diff(pts$y)^2
d = sqrt(dx+dy)
return(c(0,cumsum(d)))
}
| db7ffaa6035336ac55548a7ea05d6ad5386973a8 | [
"Markdown",
"R",
"RMarkdown"
] | 10 | R | barryrowlingson/geoutils | 700865e9d103f63f39bebfe52ed6523754e176e7 | 2aeae86d3383ac8dcdc04ca5c79ee1bff9b4dcf4 | |
refs/heads/master | <file_sep>package com.opensource.androidrepository.ui.custom;
import android.app.Activity;
import android.graphics.drawable.ColorDrawable;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.PopupWindow;
import android.widget.RelativeLayout;
import com.opensource.androidrepository.R;
/**
* Created by Administrator on 2016/10/24.
*/
public class MenuPopupWindow extends PopupWindow implements View.OnClickListener{
private View view;
private OnItemClickListener mListener;
public MenuPopupWindow(Activity context, OnItemClickListener mListener){
LayoutInflater inflater = LayoutInflater.from(context);
view = inflater.inflate(R.layout.layout_popup_menu, null);
this.mListener = mListener;
this.setContentView(view);
this.setWidth(RelativeLayout.LayoutParams.WRAP_CONTENT);
//设置SelectPicPopupWindow弹出窗体的高
this.setHeight(RelativeLayout.LayoutParams.WRAP_CONTENT);
//设置SelectPicPopupWindow弹出窗体可点击
this.setFocusable(false);
this.setOutsideTouchable(true);
this.setBackgroundDrawable(new ColorDrawable(0x00000000));
view.findViewById(R.id.lay_share).setOnClickListener(this);
view.findViewById(R.id.lay_inform).setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()){
case R.id.lay_share:
mListener.onItemClick(this, 1);
break;
case R.id.lay_inform:
mListener.onItemClick(this, 2);
break;
}
}
public interface OnItemClickListener{
void onItemClick(PopupWindow popupWindow, int position);
}
}
<file_sep>package com.opensource.androidrepository.util;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.Canvas;
import android.graphics.Matrix;
import android.graphics.Paint;
import android.graphics.PorterDuff;
import android.graphics.PorterDuffXfermode;
import android.graphics.Rect;
import android.graphics.RectF;
import android.os.Environment;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
/**
* Various bitmap utilities
*
*/
public class BitmapUtils {
/**
* Resize a bitmap
*
* @param input
* @param destWidth
* @param destHeight
* @return
* @throws OutOfMemoryError
*/
public static Bitmap resizeBitmap(final Bitmap input, int destWidth, int destHeight ) throws OutOfMemoryError {
return resizeBitmap( input, destWidth, destHeight, 0 );
}
/**
* Resize a bitmap object to fit the passed width and height
*
* @param input
* The bitmap to be resized
* @param destWidth
* Desired maximum width of the result bitmap
* @param destHeight
* Desired maximum height of the result bitmap
* @return A new resized bitmap
* @throws OutOfMemoryError
* if the operation exceeds the available vm memory
*/
public static Bitmap resizeBitmap(final Bitmap input, int destWidth, int destHeight, int rotation ) throws OutOfMemoryError {
int dstWidth = destWidth;
int dstHeight = destHeight;
final int srcWidth = input.getWidth();
final int srcHeight = input.getHeight();
if ( rotation == 90 || rotation == 270 ) {
dstWidth = destHeight;
dstHeight = destWidth;
}
boolean needsResize = false;
float p;
if ( ( srcWidth > dstWidth ) || ( srcHeight > dstHeight ) ) {
needsResize = true;
if ( ( srcWidth > srcHeight ) && ( srcWidth > dstWidth ) ) {
p = (float) dstWidth / (float) srcWidth;
dstHeight = (int) ( srcHeight * p );
} else {
p = (float) dstHeight / (float) srcHeight;
dstWidth = (int) ( srcWidth * p );
}
} else {
dstWidth = srcWidth;
dstHeight = srcHeight;
}
if ( needsResize || rotation != 0 ) {
Bitmap output;
if ( rotation == 0 ) {
output = Bitmap.createScaledBitmap(input, dstWidth, dstHeight, true);
} else {
Matrix matrix = new Matrix();
matrix.postScale( (float) dstWidth / srcWidth, (float) dstHeight / srcHeight );
matrix.postRotate( rotation );
output = Bitmap.createBitmap(input, 0, 0, srcWidth, srcHeight, matrix, true);
}
return output;
} else
return input;
}
public static void saveBitmap(Bitmap mBitmap, String dir, String fileName) {
String savePath = "";
// 判断是否挂载了SD卡
String storageState = Environment.getExternalStorageState();
if (storageState.equals(Environment.MEDIA_MOUNTED)) {
savePath = Environment.getExternalStorageDirectory().getAbsolutePath() + "/RecordVideo/" + dir;// 存放照片的文件夹
File savedir = new File(savePath);
if (!savedir.exists()) {
savedir.mkdirs();
}
}
// 没有挂载SD卡,无法保存文件
if (isEmpty(savePath)) {
// Toast.makeText(context, "无法保存照片,请检查SD卡是否挂载", Toast.LENGTH_SHORT).show();
return;
}
File f = new File(savePath, fileName);
FileOutputStream fOut = null;
try {
fOut = new FileOutputStream(f);
mBitmap.compress(Bitmap.CompressFormat.JPEG, 100, fOut);
mBitmap.recycle();
fOut.flush();
fOut.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public static boolean isEmpty(String input) {
if (input == null || "".equals(input))
return true;
for (int i = 0; i < input.length(); i++) {
char c = input.charAt(i);
if (c != ' ' && c != '\t' && c != '\r' && c != '\n') {
return false;
}
}
return true;
}
public static Bitmap getRoundedCornerBitmap(Bitmap bitmap) {
Bitmap outBitmap = Bitmap.createBitmap(bitmap.getWidth(),
bitmap.getHeight(), Bitmap.Config.ARGB_8888);
Canvas canvas = new Canvas(outBitmap);
final int color = 0xff424242;
final Paint paint = new Paint();
final Rect rect = new Rect(0, 0, bitmap.getWidth(), bitmap.getHeight());
final RectF rectF = new RectF(rect);
final float roundPX = bitmap.getWidth() / 2;
paint.setAntiAlias(true);
canvas.drawARGB(0, 0, 0, 0);
paint.setColor(color);
canvas.drawRoundRect(rectF, roundPX, roundPX, paint);
paint.setXfermode(new PorterDuffXfermode(PorterDuff.Mode.SRC_IN));
canvas.drawBitmap(bitmap, rect, rect, paint);
return outBitmap;
}
/**
* 缩放图片
*
* @param bitmap
* @param zf
* @return
*/
public static Bitmap zoom(Bitmap bitmap, float zf) {
Matrix matrix = new Matrix();
matrix.postScale(zf, zf);
return Bitmap.createBitmap(bitmap, 0, 0, bitmap.getWidth(),
bitmap.getHeight(), matrix, true);
}
/**
* 缩放图片
*
* @param bitmap
* @param hf
* @return
*/
public static Bitmap zoom(Bitmap bitmap, float wf, float hf) {
Matrix matrix = new Matrix();
matrix.postScale(wf, hf);
return Bitmap.createBitmap(bitmap, 0, 0, bitmap.getWidth(),
bitmap.getHeight(), matrix, true);
}
/**
* 图片圆角处理
*
* @param bitmap
* @param roundPX
* @return
*/
public static Bitmap getRCB(Bitmap bitmap, float roundPX) {
// RCB means
// Rounded
// Corner Bitmap
Bitmap dstbmp = Bitmap.createBitmap(bitmap.getWidth(),
bitmap.getHeight(), Bitmap.Config.ARGB_8888);
Canvas canvas = new Canvas(dstbmp);
final int color = 0xff424242;
final Paint paint = new Paint();
final Rect rect = new Rect(0, 0, bitmap.getWidth(), bitmap.getHeight());
final RectF rectF = new RectF(rect);
paint.setAntiAlias(true);
canvas.drawARGB(0, 0, 0, 0);
paint.setColor(color);
canvas.drawRoundRect(rectF, roundPX, roundPX, paint);
paint.setXfermode(new PorterDuffXfermode(PorterDuff.Mode.SRC_IN));
canvas.drawBitmap(bitmap, rect, rect, paint);
return dstbmp;
}
public static Bitmap getBitmapFromFilePath(String filePath) {
try {
return BitmapFactory.decodeFile(filePath);
} catch (OutOfMemoryError e) {
e.printStackTrace();
}
return null;
}
public static Bitmap getBitmapFromFilePath(String filePath, int width, int height) {
BitmapFactory.Options opts = null;
if (width > 0 && height > 0) {
opts = new BitmapFactory.Options();
opts.inJustDecodeBounds = true;
BitmapFactory.decodeFile(filePath, opts);
// 计算图片缩放比例
final int minSideLength = Math.min(width, height);
opts.inSampleSize = computeSampleSize(opts, minSideLength,
width * height);
opts.inJustDecodeBounds = false;
opts.inInputShareable = true;
opts.inPurgeable = true;
}
try {
return BitmapFactory.decodeFile(filePath, opts);
} catch (OutOfMemoryError e) {
e.printStackTrace();
}
return null;
}
public static Bitmap getBitmapFromFile(File dst, int width, int height) {
if (null != dst && dst.exists()) {
BitmapFactory.Options opts = null;
if (width > 0 && height > 0) {
opts = new BitmapFactory.Options();
opts.inJustDecodeBounds = true;
BitmapFactory.decodeFile(dst.getPath(), opts);
// 计算图片缩放比例
final int minSideLength = Math.min(width, height);
opts.inSampleSize = computeSampleSize(opts, minSideLength,
width * height);
opts.inJustDecodeBounds = false;
opts.inInputShareable = true;
opts.inPurgeable = true;
}
try {
return BitmapFactory.decodeFile(dst.getPath(), opts);
} catch (OutOfMemoryError e) {
e.printStackTrace();
}
}
return null;
}
public static int computeSampleSize(BitmapFactory.Options options,
int minSideLength, int maxNumOfPixels) {
int initialSize = computeInitialSampleSize(options, minSideLength,
maxNumOfPixels);
int roundedSize;
if (initialSize <= 8) {
roundedSize = 1;
while (roundedSize < initialSize) {
roundedSize <<= 1;
}
} else {
roundedSize = (initialSize + 7) / 8 * 8;
}
return roundedSize;
}
private static int computeInitialSampleSize(BitmapFactory.Options options,
int minSideLength, int maxNumOfPixels) {
double w = options.outWidth;
double h = options.outHeight;
int lowerBound = (maxNumOfPixels == -1) ? 1 : (int) Math.ceil(Math
.sqrt(w * h / maxNumOfPixels));
int upperBound = (minSideLength == -1) ? 128 : (int) Math.min(Math
.floor(w / minSideLength), Math.floor(h / minSideLength));
if (upperBound < lowerBound) {
// return the larger one when there is no overlapping zone.
return lowerBound;
}
if ((maxNumOfPixels == -1) && (minSideLength == -1)) {
return 1;
} else if (minSideLength == -1) {
return lowerBound;
} else {
return upperBound;
}
}
public static Bitmap addPadding(Bitmap bmp, int color) {
if (bmp == null) {
return null;
}
int biggerParam = Math.max(bmp.getWidth(), bmp.getHeight());
Bitmap bitmap = Bitmap.createBitmap(biggerParam, biggerParam, bmp.getConfig());
Canvas canvas = new Canvas(bitmap);
canvas.drawColor(color);
int top = bmp.getHeight() > bmp.getWidth() ? 0 : (bmp.getWidth() - bmp.getHeight())/2;
int left = bmp.getWidth() > bmp.getHeight() ? 0 : (bmp.getHeight() - bmp.getWidth())/2;
canvas.drawBitmap(bmp, left, top, null);
return bitmap;
}
public static byte[] bmpToByteArray(final Bitmap bmp, final boolean needRecycle) {
ByteArrayOutputStream output = new ByteArrayOutputStream();
bmp.compress(Bitmap.CompressFormat.PNG, 100, output);
if (needRecycle) {
bmp.recycle();
}
byte[] result = output.toByteArray();
try {
output.close();
} catch (Exception e) {
e.printStackTrace();
}
return result;
}
}
<file_sep>package com.opensource.androidrepository.ui.activity;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import com.opensource.androidrepository.R;
public class DrawFocusRectActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_draw_focus_rect);
}
}
<file_sep># mine-android-repository
这个是我平时项目中一些自己封装的代码库,如自定义控件、dialog、小模块等。
使用正则表达式来匹配 文本,然后高亮显示,点击跳到相应的页面。 如微博为的 @某人, #新闻热搜# 话题。
平时的学习资源、经典案例
https://github.com/xiaoxiaoqingyi/mine-study-resource
<file_sep>package com.opensource.androidrepository.ui.activity;
import android.app.Dialog;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.PopupWindow;
import android.widget.TextView;
import android.widget.Toast;
import com.opensource.androidrepository.R;
import com.opensource.androidrepository.ui.custom.CommomDialog;
import com.opensource.androidrepository.ui.custom.MenuPopupWindow;
import com.opensource.androidrepository.ui.custom.ShareDialog;
import com.opensource.androidrepository.util.Utils;
public class AllDialogActivity extends AppCompatActivity implements View.OnClickListener{
private TextView menu;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_all_dialog);
initView();
}
private void initView(){
setTitle("弹窗");
findViewById(R.id.txt).setOnClickListener(this);
findViewById(R.id.share).setOnClickListener(this);
menu =(TextView)findViewById(R.id.menu);
menu.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()){
case R.id.txt:
//弹出提示框
new CommomDialog(this, R.style.dialog, "您确定删除此信息?", new CommomDialog.OnCloseListener() {
@Override
public void onClick(Dialog dialog, boolean confirm) {
if(confirm){
Toast.makeText(AllDialogActivity.this,"点击确定", Toast.LENGTH_SHORT).show();
dialog.dismiss();
}
}
})
.setTitle("提示").show();
break;
case R.id.menu:
MenuPopupWindow menuPopupWindow = new MenuPopupWindow(this, new MenuPopupWindow.OnItemClickListener() {
@Override
public void onItemClick(PopupWindow popupWindow, int position) {
popupWindow.dismiss();
}
});
menuPopupWindow.showAsDropDown(menu, -200, 40);
break;
case R.id.share:
new ShareDialog(AllDialogActivity.this, R.style.dialog, new ShareDialog.OnItemClickListener() {
@Override
public void onClick(Dialog dialog, int position) {
dialog.dismiss();
switch (position){
case 1:
Utils.toast(AllDialogActivity.this,"微信好友");
break;
case 2:
Utils.toast(AllDialogActivity.this,"朋友圈");
break;
case 3:
Utils.toast(AllDialogActivity.this,"QQ");
break;
case 4:
Utils.toast(AllDialogActivity.this,"微博");
break;
}
}
}).show();
break;
}
}
}
| c1b50cf60976f6d4066dab91c7ccb3dde0a12f6d | [
"Markdown",
"Java"
] | 5 | Java | xiaoxiaoqingyi/mine-android-repository | 91263be16da00369205f08e802db02b74d466d47 | f797b168ce461e5647d58919cfd096ca1f5e0fdf | |
refs/heads/master | <file_sep>$( window ).scroll(function() {
var wScroll = $(this).scrollTop();
$('.logo').css({
'transform' : 'translate(0px, '+ wScroll /2.5+'%)'
})
if(wScroll > $('.beer').offset().top - 600){
$('.beer-bottle').each(function(){
$('.beer-bottle').addClass('is-showing');
})
}
});
| 1930a349accba819539470d2625da8142665ac0a | [
"JavaScript"
] | 1 | JavaScript | jk21635/GroveCityBrewing | 519b32e4af8c1c131203525abf3208e09b7dd0a3 | 066011b3f61d8d2c22b17d1e91e59e7585ea4c9a | |
refs/heads/master | <repo_name>aml007/ciblue<file_sep>/application/views/news/success.php
<h2>The record was created successfully!</h2>
| a6cf64da918d3b9a14b6bb1568b0e1ea2de18518 | [
"PHP"
] | 1 | PHP | aml007/ciblue | ce73719c8ac7c4d08ce86dabeb30c61ab085e2e3 | 81fa302bcf4a4f8660c3ec6685adb497bed7b023 | |
refs/heads/master | <repo_name>bellaheidii/Grace-Hoper<file_sep>/client/build/precache-manifest.c0d6e1b47873705f6a5683506b6a9c32.js
self.__precacheManifest = [
{
"revision": "42ac5946195a7306e2a5",
"url": "/static/js/runtime~main.a8a9905a.js"
},
{
"revision": "9597a14fe210b2f57a0a",
"url": "/static/js/main.0e61a698.chunk.js"
},
{
"revision": "b5c5beb43fab1ff7f479",
"url": "/static/js/2.ec295295.chunk.js"
},
{
"revision": "59a4c23f30beb26ad21357f618efc8b4",
"url": "/index.html"
}
];<file_sep>/scripts/seedDB.js
const mongoose = require("mongoose");
const db = require("../models");
// This file empties the Books collection and inserts the books below
mongoose.connect(
process.env.MONGODB_URI ||
"mongodb://localhost/grace-hoper"
);
const productSeed = [
{
catagory: "Clothing",
productName: "<NAME>",
condition:"Good",
date: new Date(Date.now())
},
{
catagory: "Clothing",
productName: "Jordan shoes",
condition: "Excellent",
date: new Date(Date.now())
},
{
catagory: "Electronics",
productName: "Samsung Tv",
condition: "Good",
date: new Date(Date.now())
},
{
catagory: "Electronics",
productName: "Iphone 8 plus",
condition: "Excellent",
date: new Date(Date.now())
},
{
catagory: "Furniture",
productName: "Mattresses",
condition:"Good",
date: new Date(Date.now())
},
{
catagory: "Housewares",
productName: "Microwave",
condition: "Excellent",
date: new Date(Date.now())
},
{
catagory: "Beauty",
productName: "Clinique",
condition: "Good",
date: new Date(Date.now())
},
{
catagory: "Electronics",
productName: "Iphone 8 plus",
condition: "Excellent",
date: new Date(Date.now())
},
{
catagory: "Furniture",
productName: "Luca Modern Sofa",
condition:"Good",
date: new Date(Date.now())
},
{
catagory: "Clothing",
productName: "Nike pants",
condition: "Excellent",
date: new Date(Date.now())
},
{
catagory: "Electronics",
productName: "kodak Camera",
condition: "Good",
date: new Date(Date.now())
},
{
catagory: "Housewares",
productName: "Refrigerators",
condition: "Excellent",
date: new Date(Date.now())
},
];
db.Products
.remove({})
.then(() => db.Products.collection.insertMany(productSeed))
.then(data => {
console.log(data.result.n + " records inserted!");
process.exit(0);
})
.catch(err => {
console.error(err);
process.exit(1);
});
<file_sep>/routes/api/index.js
const path = require("path");
const router = require("express").Router();
const productRoutes = require("./products");
const customerRoutes = require("./customer");
// Book routes
router.use("/books", productRoutes);
// Google Routes
router.use("/profile", customerRoutes);
// For anything else, render the html page
router.use(function(req, res) {
res.sendFile(path.join(__dirname, "../../client/build/index.html"));
});
module.exports = router;<file_sep>/models/customers.js
const mongoose = require("mongoose");
const Schema = mongoose.Schema;
const customersSchema = new Schema({
firstName: { type: String, required: true },
lastName: { type: String, required: true },
email:{ type: String, required: true} ,
password: {type: String, required: true},
date: { type: Date, default: Date.now },
products: [
{
type: Schema.Types.ObjectId,
ref: "products"
}
]
});
const Customers = mongoose.model("Customers", customersSchema);
module.exports = Customers;<file_sep>/client/src/App.js
import React from 'react';
import { BrowserRouter as Router, Route, Switch } from "react-router-dom";
//import './App.css';
import Footer from './Containers/FooterComponent';
import NavBar from './Containers/NavBarComponent';
import LogIn from "./Pages/LogIn";
import SignUp from "./Pages/SignUp";
import Cart from "./Pages/Cart";
import Home from "./Pages/Home";
import Beauty from "./Pages/Beauty";
import Books from "./Pages/Books";
import ChildrenToys from "./Pages/ChildrenToys";
import Clothes from "./Pages/Clothes";
import Electronics from "./Pages/Electronics";
import Jewelry from "./Pages/Jewelry";
import Movies from "./Pages/Movies";
import Sport from "./Pages/Sport";
import GardenTools from './Pages/GardenTools';
import Search from './Pages/Search';
function App() {
return (
<div>
<Router>
<div>
<NavBar />
<Switch>
<Route exact path="/" component={Home} />
<Route exact path="/LogIn" component={LogIn} />
<Route exact path="/SignUp" component={SignUp} />
<Route exact path="/cart" component={Cart} />
<Route exact path="/Search" component={Search} />
<Route exact path="/Beauty" component={Beauty} />
<Route exact path="/Books" component={Books} />
<Route exact path="/ChildrenToys" component={ChildrenToys} />
<Route exact path="/Clothes" component={Clothes} />
<Route exact path="/Electronics" component={Electronics} />
<Route exact path="/Jewelry" component={Jewelry} />
<Route exact path="/Movies" component={Movies} />
<Route exact path="/Sport" component={Sport} />
<Route exact path="/GardenTools" component={GardenTools} />
</Switch>
<Footer />
</div>
</Router>
</div>
);
}
export default App;
<file_sep>/models/products.js
const mongoose = require("mongoose");
const Schema = mongoose.Schema;
const productsSchema = new Schema({
catagory: { type: String, required: true },
productName: { type: String, required: true },
condition: { type: String, required: true} ,
// image: { data: Buffer, type: String, required: true },
date: { type: Date, default: Date.now }
});
const Products = mongoose.model("Book", productsSchema);
module.exports = Products; | 5920ae1073b122d1bc177ebb3560d526bca9ad45 | [
"JavaScript"
] | 6 | JavaScript | bellaheidii/Grace-Hoper | de6fd020796ec6eb8c45b1b0ffee111a82f57baa | a55750ce4b9c7941841378e2fcf12c438e22062b | |
refs/heads/master | <repo_name>SibeleNepomuceno/Projeto-2<file_sep>/src/pi/PI.java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package pi;
import com.sun.org.apache.bcel.internal.generic.SWITCH;
import java.sql.Connection;
import java.sql.Date;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.Scanner;
import java.util.Vector;
import modelo.agendamento;
import pi.dao.Conexao;
import pi.dao.clienteDao;
import modelo.cliente;
import modelo.funcionario;
import modelo.servico;
import modelo.status;
import modelo.unidade;
import pi.dao.agendamentoDao;
import pi.dao.funcionarioDao;
import pi.dao.servicoDao;
import pi.dao.statusDao;
import pi.dao.unidadeDao;
/**
*
* @author Alessandra
*/
public class PI {
static int menu() {
Scanner leitor = new Scanner(System.in);
System.out.println(" ");
System.out.println(" >>>>>>>> Agendamento Eletrônico <<<<<<<<");
System.out.println("");
System.out.println(" ++++++ Esmalteria - Com que Cor? ++++++");
System.out.println(" ");
System.out.println(" 1 - Agendar "); // verificar agendas ou efetuar agendamentos
System.out.println(" 2 - Relatorios"); //
System.out.println(" 3 - Cadastro "); // editar, excluir, visualizar
System.out.println(" 0 - Sair ");
System.out.println(" ");
int op = leitor.nextInt();
return op;
}
// menu para editar, excluir e visualizar o cadastro
static int submenu() {
Scanner leitor = new Scanner(System.in);
System.out.println(" Escolha a opção ");
System.out.println(" 1 - Editar ");
System.out.println(" 2 - Excluir ");
System.out.println(" 3 - Visualizar ");
int op3 = leitor.nextInt();
return op3;
}
// menu que vai diferenciar em qual cadastro a pessoa vai gerenciar, se é cliente ou funcionario
static int submenu1() {
Scanner leitor = new Scanner(System.in);
System.out.println(" Escolha a opção que deseja gerenciar ");
System.out.println(" 1 - Cliente ");
System.out.println(" 2 - Funcionário ");
int op2 = leitor.nextInt();
return op2;
}
static int relatorios() {
Scanner leitor = new Scanner(System.in);
System.out.println(" Informe qual relatório deseja consultar ");
System.out.println(" 1 - fluxo de atendimentos na unidade. ");
System.out.println(" 2 - fluxo de atendimentos por funcionário");
System.out.println(" 3 - fluxo de serviço prestado ");
System.out.println(" 4 - total de clientes que participaram da promoção ");
System.out.println(" 5 - total de frequencia de cada cliente ");
int opRelatorio = leitor.nextInt();
return opRelatorio;
}
static int cadastro() {
Scanner leitor = new Scanner(System.in);
System.out.println(" 1 - Realizar Agendamento ");
System.out.println(" 2 - Alterar Agendamento ");
System.out.println(" 3 - Visualizar Agendamento ");
int opCadastro = leitor.nextInt();
return opCadastro;
}
public static void main(String[] args) throws SQLException {
Scanner leitor = new Scanner(System.in);
//leitor.nextLine();
// conectar com o banco de dados
Connection conn = Conexao.conectar();
// importar as classes de conexão com banco de dados
clienteDao cli = new clienteDao(conn);
agendamentoDao agend = new agendamentoDao(conn);
unidadeDao uni = new unidadeDao(conn);
funcionarioDao fun = new funcionarioDao(conn);
servicoDao ser = new servicoDao(conn);
statusDao status = new statusDao(conn);
// importa as classes
cliente clie = new cliente();
agendamento agendar = new agendamento();
unidade unidade1 = new unidade();
funcionario func = new funcionario();
servico serv = new servico();
status sta = new status();
int op; //menu principal
do {
op = menu();
switch (op) {
case 1:
int opCadastro = cadastro();
switch (opCadastro) {
case 1: // efetuar o agendamento
//encontrar as unidades no banco de dados
System.out.println(" Informe qual unidade deseja realizar o serviço ");
System.out.println(" ");
uni.buscar(unidade1);
int unidade = leitor.nextInt();
agendar.setIdUnidade(unidade);
// agend.insere(agendar); //insere no banco - agendamento
// escolher funcionario
System.out.println(" Digite o código da Funcionário(a):");
System.out.println(" ");
fun.pesquisarPorID(unidade); //irá chamar a profissional da unidade digitada anteriormente
int funcionario = leitor.nextInt();
agendar.getIdFuncionario(); //guarda na classe o valor (ID do funcionario)
//recebendo o nome do cliente
//informa o cliente
System.out.println(" Informe o cliente");
System.out.println(" ");
System.out.println(" 1 - Por nome - caso não possua cadastro "); //por nome caso o cliente não esteja cadastrado
System.out.println(" 2 - Por código "); //codigo do cadastro
int escolha = leitor.nextInt();
if (escolha == 1) { //se a opcao for 1, nome será guardado na variavel de nome da classe agendamento
System.out.println(" Digite o nome: ");
String nomeCliente = leitor.next();
agendar.setNomeCliente(nomeCliente);
} else if (escolha == 2) {
System.out.println(" Digite o código: ");
int idCliente = leitor.nextInt();
agendar.setIdCliente(idCliente);
}
System.out.println(" ");
System.out.println(" Digite o código do serviço que deseja ");
System.out.println(" ");
ser.buscar(serv);
int servico = leitor.nextInt();
agendar.setIdServico(servico); // inclui na classe o valor recebido na variavel
System.out.println(" ");
//insere a data
System.out.println(" Informe a data ");
System.out.println(" ");
String data = leitor.next();
agendar.setDataAgendamento(data);//insere a data na classe agendamento
System.out.println(" ");
System.out.println(" Informe o horário inicial - cada serviço terá 30 minutos para realização ");
System.out.println(" ");
String horaInicial = leitor.next();
agendar.setHoraInicial(horaInicial);
System.out.println(" ");
System.out.println(" Informe o horário final - cada serviço terá 30 minutos para realização ");
System.out.println(" ");
String horaFinal = leitor.next();
agendar.setHoraFinal(horaFinal);
//verificação se a cliente esta participando da promoção
System.out.println(" ");
System.out.println(" Digite a opção : ");
System.out.println(" Participa da Promoção ? ");
System.out.println(" ");
System.out.println(" 1 - Sim ");
System.out.println(" 2 - Não ");
int opPromocao = leitor.nextInt();
//compara se participa da promocao ou não
if (opPromocao == 1) {
boolean promocao = true;
//guarda na classe a resposta
agendar.setPromocao(promocao);
} else {
boolean promocao = false;
//guarda na classe a resposta
agendar.setPromocao(promocao);
}
System.out.println(" Valor ");
System.out.println(" ");
Double val = leitor.nextDouble();
agendar.setValor(val);
//informa o status do agendamento
System.out.println(" ");
System.out.println(" Digite o código do Status ");
System.out.println(" ");
status.buscar(sta);
int idStatus = leitor.nextInt();
agendar.setIdStatus(idStatus);
//fila de espera
System.out.println(" Participa da Fila de Espera?");
System.out.println(" ");
System.out.println(" 1 - Sim ");
System.out.println(" 2 - Não ");
int opFila = leitor.nextInt();
//compara se participa da promocao ou não
if (opFila == 1) {
boolean fila = true;
//guarda na classe a resposta
agendar.setFilaEspera(fila);
} else {
boolean fila = false;
//guarda na classe a resposta
agendar.setFilaEspera(fila);
}
System.out.println(" Agendamento Realizado !!! ");
agend.insere(agendar); //insere as informações na tabela agendamento do banco de dados
break;
case 2:
break;
case 3:
System.out.println(" ");
System.out.println(" Código do cliente | Código da unidade | Código do serviço | Código do status | Código do agendamento | Hora Inicial | Hora Final | Fila de Espera | Promoção | Data do agendamento");
System.out.println(" ");
agend.buscar(agendar);
System.out.println(" ");
break;
}
break;
case 2:
int opRelatorio = relatorios();
switch (opRelatorio) {
case 1:
case 2:
case 3:
case 4:
case 5:
}
break;
case 3:
int op2 = submenu1(); //submenu para gerenciar cadastro - escolhe qual cadastro gerenciar
switch (op2) {
case 1: //cliente
int opcaoCliente = submenu(); // editar, excluir ou visualizar - cliente
switch (opcaoCliente) {
case 1:
System.out.println(" Informe qual cliente deseja editar ");// condição, precisa ver no banco qual chave primaria
cli.atualizar(clie);
break;
case 2:
System.out.println(" Informe qual codigo do cliente deseja excluir ");
//comparar se possui nome para excluir ******
int idApagar = leitor.nextInt();
cli.apagar(idApagar);
System.out.println(" Cadastro apagado com sucesso !! ");
break;
case 3:
System.out.println(" ");
System.out.println(" Código | Nome | CPF | RG | Telefone | Celular |");
System.out.println(" ");
cli.buscar(clie);
System.out.println(" ");
break;
}
break;
case 2: //funcionario
int opcaoFuncionario = submenu();// editar, excluir ou visualizar - Funcionario
switch (opcaoFuncionario) {
case 1:
System.out.println(" Informe qual o código do funcionário que deseja editar ");// condição, precisa ver no banco qual chave primaria
break;
case 2:
System.out.println(" Informe qual o código do funcionário que deseja excluir ");// condição, precisa ver no banco qual chave primaria
int idApagar = leitor.nextInt();
fun.apagar(idApagar); //passa o id para a funcao apagar da classe funcionario que faz conexão com DB
System.out.println(" Cadastro apagado com sucesso !! ");
break;
case 3:
System.out.println(" ");
System.out.println(" Código do Funcionário | Código da Unidade | Nome do Funcionario | CPF | RG | Cargo |");
System.out.println(" ");
fun.buscar(func);
System.out.println(" ");
break;
}
}
break;
default:
System.out.println(
" Opção invalida !!! ");
}
/*System.out.println(" Nome: ");
String nome = leitor.nextLine();
System.out.println(" CPF: ");
String cpf = leitor.nextLine();
System.out.println(" RG: ");
String rg = leitor.nextLine();
System.out.println(" Telefone: ");
String tel = leitor.nextLine();
System.out.println(" Celular: ");
String cel = leitor.nextLine();
clie.setNome(nome);
clie.setRg(rg);
clie.setCpf(cpf);
clie.setCelular(cel);
clie.setTelefone(tel);
cli.insere(clie);*/
} while (op != 0);
}
}
| 42e809cb0d67325a87ebb17ce24da06cb547962c | [
"Java"
] | 1 | Java | SibeleNepomuceno/Projeto-2 | 404f985df45cdbed4677567eeae5149023046858 | 3ccfe29d24471d4d18a0b8fac569e10c81d7e331 | |
refs/heads/master | <file_sep>#!/bin/bash
if [[ $UID != 0 ]]; then
echo "Please run this script with sudo:"
echo "sudo $0 $*"
exit 1
fi
echo -e "Please specify the hostname for this machine: "
read hname
echo -e "And the domain? "
read domain
echo -e "Adding hostname to /etc/hostname... \n"
hostnamectl set-hostname "$hname"
sleep 0.5s
echo -e "Replacing /etc/hosts... \n"
sed -i ./configs/hosts s/HNAME/$hname
sed -i ./configs/hosts s/DOMAIN/$domain
cat ./configs/hosts > /etc/hosts
echo -e "Configuring git variables... \n"
git config --global color.ui auto
git config --global user.name alexfornuto
git config --global user.email <EMAIL>
sleep .5s
echo -e "Copying the scripts directory... \n"
mv .scripts ~/
sleep 1.5s
echo -e "Copying Screen config file into ~ ... \n"
cp configs/.screenrc ~/.screenrc
sleep .5s
echo -e "making the .ssh dir, if not already there... \n"
mkdir -p ~/.ssh
sleep .5s
echo -e "Adding the list of public keys from github into the authorized_keys file for this user... \n"
curl https://github.com/alexfornuto.keys >> ~/.ssh/authorized_keys
sleep 1.5s
echo -e "Adding aliases and colors into .bashrc... \n"
cat configs/.bashrc >> ~/.bashrc
sleep .5s
echo -e "sourcing bash profile... \n"
source ~/.bashrc
sleep .5s
echo -e "Done! \n"
<file_sep>bash-setup
==========
Configurations and Scripts I use on new machines. This is for personal use, but feel free to copy it if you like some of this automation. If you do, be sure to remove my public keys from your branch, and edit the Git configuration commands.
This repo should be pulled by running the [first](https://gist.github.com/alexfornuto/228fe6e1280648b37534) script from gist. You can do this in one line:
```
curl -s https://gist.githubusercontent.com/alexfornuto/228fe6e1280648b37534/raw/69c42f78ab145a742a1e672d6a3e1c7da34a502d/first.sh | bash
```
The full contents of that script are below:
```
#!/bin/bash
echo -e "updating the timezone... \n"
sudo cp /usr/share/zoneinfo/America/New_York /etc/localtime
sleep 1
echo -e "Updating, upgrading, installing packages... \n"
sudo apt-get update
sudo apt-get upgrade -y
sudo apt-get install -y git ruby rake
sleep 1
echo -e "Configuring git variables... \n"
git config --global color.ui auto
git config --global user.name alexfornuto
git config --global user.email <EMAIL>
sleep 1
echo -e "cloning bash-setup... \n"
git clone https://github.com/alexfornuto/bash-setup.git
```
The CentOS version: https://gist.github.com/alexfornuto/b61eb6301bfa5d4c6543
```
pretty much the same thing, but s/apt-get/yum/g
```
<file_sep>#!/bin/bash
# A simple script to let you unrar all archives in a set of subdirectories into your current directory.
echo "dirs?"
read dirs
echo "name?"
read name
for d in $dirs; do ( cd $d && unrar x $name ../ ); done
<file_sep>alias sprungeout="curl -F 'sprunge=<-' http://sprunge.us"
alias bigfiles="sudo du -h -d 5 / | grep '[0-9]G '"
alias updateiptables='sudo iptables-restore < /etc/iptables.firewall.rules'
alias untar='tar -zxvf'
alias showdebpacks='dpkg --get-selections | grep -v deinstall | less'
alias tarlist='tar -tvf'
PS1='\[\e[1;33m\]\u\[\e[m\] \[\e[1;34m\]\w\[\e[m\] \[\e[1;32m\]\$\[\e[m\] \[\e[1;37m\]'
source ~/.scripts/git-prompt.sh
PS1='[\u@\h \W$(__git_ps1 " (%s)")]\$'
source ~/.scripts/git-completion.bash
| 0b9e2ad10ece100342c4ab4f0780a6fc579fece5 | [
"Markdown",
"Shell"
] | 4 | Shell | alexfornuto/bash-setup | 148650d9bde6aabd7f5ebfe36a6da5c75fb8a05b | 9e3b08c51939ded09641d14bf269f1cb84622e8a | |
refs/heads/master | <repo_name>UniqueFlyingCat/jd_pc<file_sep>/js/index.js
// api start
var log = function() {
console.log.apply(console, arguments)
}
var e = function(selector) {
return document.querySelector(selector)
}
var es = function(selector) {
return document.querySelectorAll(selector)
}
var bindEle = function(element, eventName, callback) {
element.addEventListener(eventName, callback)
}
var bindSel = function(selector, eventName, callback) {
var element = document.querySelector(selector)
element.addEventListener(eventName, callback)
}
var bindAllEle = function(elements, eventName, callback) {
for (var i = 0; i < elements.length; i++) {
var e = elements[i]
bindEle(e, eventName, callback)
}
}
var bindAllSel = function(selector, eventName, callback) {
var elements = document.querySelectorAll(selector)
for (var i = 0; i < elements.length; i++) {
var e = elements[i]
bindEle(e, eventName, callback)
}
}
var toggleClass = function(element, className) {
if (element.classList.contains(className)) {
element.classList.remove(className)
} else {
element.classList.add(className)
}
}
var removeClassAll = function(className) {
var selector = "." + className
var elements = document.querySelectorAll(selector)
for (var i = 0; i < elements.length; i++) {
var e = elements[i]
e.classList.remove(className)
}
}
//api end
// start
var scroll = function() {
return {
"top": window.pageYOffset || document.body.scrollTop || document.documentElement.scrollTop,
"left": window.pageXOffset || document.body.scrollLeft || document.documentElement.scrollLeft
}
}
// scroll end
//getStyle start
var getStyle = function(element, attribute) {
if (window.getComputedStyle) {
return window.getComputedStyle(element, null)[attribute]
}
return element.currentStyle[attribute]
}
//getStyle end
// animateSlow star
var animateSlow = function(element, json, millisec, callback) {
clearInterval(element.time)
element.time = setInterval(function() {
var bool = true
for (var k in json) {
var current = parseInt(getStyle(element, k)) || 0
var step = (json[k] - current) / 10
step = step > 0 ? Math.ceil(step) : Math.floor(step)
current = current + step
element.style[k] = current + "px"
if (json[k] !== current) {
bool = false
}
}
if (bool) {
clearInterval(element.time)
if (callback) {
callback()
}
}
}, millisec)
}
// animateSlow end
//
// animateOpacity start
var animateOpacity = function(element, target, second) {
var w = second*1000
element.time = setInterval(function() {
var current = Number(getStyle(element,"opacity")) || 0
var step = Math.abs(target-current)/second/10
step = (target-current)<0?-step:step
element.style.opacity = current + step
},10)
var wait = setTimeout(function(){
clearInterval(element.time)
},w)
}
// animateOpacity end
//
//sidebar-left start
var sidebarLeft = function() {
var sidebarLeft = document.querySelector(".sidebar-left")
var height = 300
var floorArr = document.querySelectorAll(".floorArr")
var floorBoxArr = document.querySelectorAll(".floorBoxArr")
var time = null
var target = 0
var current = 0
bindEle(window, "scroll", function() {
if (scroll().top > height) {
sidebarLeft.style.display = "block"
sidebarLeft.className = "sidebar-left fixed"
}
current = scroll().top
for (var i = 0; i < floorArr.length - 1; i++) {
if ((floorBoxArr[1].offsetTop - 50 + 553 * (i + 1)) > current
&¤t > (floorBoxArr[1].offsetTop - 50 - 10 + 553 * i)) {
floorArr[i].style.background = "#B61D1D"
}else{
floorArr[i].style.background = "#918989"
}
}
})
for (var i = 0; i < floorArr.length; i++) {
floorArr[i].index = i
bindEle(floorArr[i], "click", function() {
if (this.index === 5) {
target = floorBoxArr[0].offsetTop
} else if (this.index === 0) {
target = floorBoxArr[1].offsetTop - 50
} else if (this.index === 1) {
target = floorBoxArr[1].offsetTop - 50 + 553 * 1
} else if (this.index === 2) {
target = floorBoxArr[1].offsetTop - 50 + 553 * 2
} else if (this.index === 3) {
target = floorBoxArr[1].offsetTop - 50 + 553 * 3
} else if (this.index === 4) {
target = floorBoxArr[1].offsetTop - 50 + 553 * 4
}
clearInterval(time)
time = setInterval(function() {
var step = (target - current) / 10
step = step > 0 ? Math.ceil(step) : Math.floor(step);
current = current + step
window.scrollTo(0, current)
if (Math.abs(target - current) <= Math.abs(step)) {
window.scrollTo(0, target)
clearInterval(time)
}
}, 28)
})
}
}
//sidebar-left start
// fix-toolbar start
var fixToolbar = function() {
var fixToolbar = document.querySelector(".fix-toolbar")
var height = 300
bindEle(window, "scroll", function() {
if (scroll().top > height) {
fixToolbar.style.display = "block"
fixToolbar.className = "fix-toolbar fixed"
}
current = scroll().top
})
var fixTop = document.querySelector(".fix-toolbar-top")
var time = null
var target = 0
var current = 0
bindEle(fixTop, "click", function() {
clearInterval(time)
time = setInterval(function() {
var step = (target - current) / 10
step = step > 0 ? Math.ceil(step) : Math.floor(step);
current = current + step;
window.scrollTo(0, current)
if (current === 0) {
clearInterval(time)
}
}, 28)
})
var tyArr = document.querySelectorAll(".fix-toolbar-ty")
var tyIArr = document.querySelectorAll(".fix-toolbar-ty i")
for (var i = 0; i < tyArr.length; i++) {
tyArr[i].index = i
bindEle(tyArr[i], "mouseover", function() {
tyIArr[this.index].style.background = "#B61D1D"
animateSlow(tyIArr[this.index], {
"margin-left": -51
}, 15)
})
bindEle(tyArr[i], "mouseout", function() {
tyIArr[this.index].style.background = "#7a6e6e"
animateSlow(tyIArr[this.index], {
"margin-left": 0
}, 15)
})
}
}
// fix-toolbar end
// fix-search start
var fixSearch = function() {
var fixSearch = document.querySelector(".fix-search")
var height = 260
bindEle(window, "scroll", function() {
if (scroll().top > height) {
fixSearch.style.display = "block"
fixSearch.className = "fix-search fixed"
} else {
fixSearch.style.display = "none"
fixSearch.className = "fix-search"
}
})
}
// fix-search end
// fix-banner star
var fixBanner = function() {
var img = document.querySelectorAll(".fix-banner div")
var direction = "y"
bindEle(window, "scroll", function() {
var top = scroll().top + 256
animateSlow(img[0], {
"top": top
}, 28)
animateSlow(img[1], {
"top": top
}, 28)
})
var ad = document.querySelector(".fix-banner")
bindAllSel(".fix-banner span", "click", function() {
animateOpacity(ad,0,2)
var wait = setTimeout(function(){
ad.style.display = "none"
},200)
})
}
// fix-banner end
// top-ad start
var topAd = function() {
var ad = document.querySelector(".top-ad")
bindSel(".close-ad", "click", function() {
animateOpacity(ad,0,1.5)
var wait = setTimeout(function(){
ad.style.display = "none"
},400)
})
}
//carousel start
var carousel = function() {
var timer = 1000
var change = function(i, cur) {
var len = 4
if (i == 1) {
var index = (len + cur - 1) % len
}
if (i == 2) {
var index = (cur + 1) % len
}
changeDirect(index)
}
var changeDirect = function(index) {
var element1 = e(`.img-${index}`)
var element2 = e(`.list${index}`)
var button1 = e(".carousel-button-left")
var button2 = e(".carousel-button-right")
var button3 = e("#carousel-button-prev")
var button4 = e("#carousel-button-next")
button1.dataset.list = index
button2.dataset.list = index
button3.dataset.list = index
button4.dataset.list = index
removeClassAll("active")
removeClassAll("highlight")
toggleClass(element1, "active")
toggleClass(element2, "highlight")
}
var changeAuto = function() {
var button1 = e(".carousel-button-left")
var cur = parseInt(button1.dataset.list)
var i = 2
change(i, cur)
}
bindAllSel(".carousel-button button", "click", (event) => {
var self = event.target
var index = parseInt(self.dataset.index)
var cur = parseInt(self.dataset.list)
clearInterval(time1)
change(index, cur)
time1 = setInterval(changeAuto, timer)
})
bindAllSel(".carousel-list li", "mouseover", (event) => {
var self = event.target
var index = parseInt(self.dataset.list)
clearInterval(time1)
changeDirect(index)
})
bindAllSel(".carousel-list li", "mouseout", () => {
time1 = setInterval(changeAuto, timer)
})
bindSel(".carousel-one", "mouseover", () => {
clearInterval(time1)
var button = document.querySelector(".carousel-button")
button.style.display = "block"
})
bindSel(".carousel-one", "mouseout", () => {
var button = document.querySelector(".carousel-button")
button.style.display = "none"
clearInterval(time1)
time1 = setInterval(changeAuto, timer)
})
var time1 = setInterval(changeAuto, timer)
}
//carousel end
//main start
function main() {
sidebarLeft()
fixToolbar()
fixBanner()
fixSearch()
topAd()
carousel()
}
main()
//main end
<file_sep>/README.md
# jd_pc
作品地址:https://uniqueflyingcat.github.io/jd_pc/

| 04197b43d488d4d24475ee7e0e8524c6b50fc018 | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | UniqueFlyingCat/jd_pc | 0903c619d47e68fc365e2d6f2679e6fb6bc6347e | aac19ab389fbe9f089f1615d9640145766e73733 | |
refs/heads/master | <repo_name>cperera/sokoban<file_sep>/sokoban.py
#! /usr/local/bin/python3
import copy
import os
from unittest.mock import MagicMock
# We decided to use letters for input
class Sokoboard:
moves = {
"n": [-1,0],
"s": [1,0],
"e": [0,1],
"w": [0,-1]
}
def __init__(self,raw):
self.board = []
self.player = [0,0]
start = 0
row_num = 0
line = raw.find('\n')
while line != -1:
row = raw[start:line]
player_x = row.find("@")
if player_x > 0:
self.player = [row_num,player_x]
else:
player_x = row.find("+")
if player_x > 0:
self.player = [row_num,player_x]
row.find("+") != -1
self.board = self.board + [[char for char in row]]
start = line + 1
row_num += 1
line = raw.find('\n',start)
self.board += [[char for char in raw[start:]]]
def is_valid_move(self, direction):
next_location = add_vectors(self.player, self.moves[direction])
if (self.get_square_at_location(next_location) == "$"):
next_next_location = add_vectors(next_location, self.moves[direction])
if self.get_square_at_location(next_next_location) == "$":
return False
elif self.get_square_at_location(next_next_location) == "#":
return False
return self.get_square_at_location(next_location) != "#"
def get_square_at_location(self, location):
return self.board[location[0]][location[1]]
def won(self):
for row in self.board[1:-1]:
if "$" in row:
return False
return True
def move(self, direction):
newBoard = copy.deepcopy(self.board)
player_destination = "@"
player_origin = " "
if self.get_square_at_location(self.player) == "+":
player_origin = "."
self._set_location(newBoard, self.player, player_origin)
next_location = add_vectors(self.player, self.moves[direction])
if self.get_square_at_location(next_location) == "$":
next_next_location = add_vectors(next_location, self.moves[direction])
box_destination = "$"
if self.get_square_at_location(next_next_location) == ".":
box_destination = "*"
self._set_location(newBoard, next_next_location, box_destination)
elif self.get_square_at_location(next_location) == ".":
player_destination = "+"
self._set_location(newBoard, next_location, player_destination)
return Sokoboard.from_board(newBoard)
def _set_location(self, board, location, value):
board[location[0]][location[1]] = value
return board
def to_string(self):
return "\n".join(["".join(row) for row in self.board])
@classmethod
def from_board(cls, board):
return cls("\n".join(["".join(row) for row in board]))
# When calls, prompts user for input and returns a valid (but not necessarily legal) direction
def next_step(board, message = ""):
os.system('cls' if os.name == 'nt' else 'clear')
print(board.to_string())
if message:
print(message)
test = input("Please enter a direction or command. Commands are Q for quit, R for restart \nDirection is one of n, s, e, w (north, south, east, west)\n")
if (not test in ["n", "s", "e", "w", "R", "Q"]): # directions, restart, quit
return next_step(board, message = "That's not a valid input.")
return test
def add_vectors(vector1, vector2):
return [x + vector2[i] for (i, x) in enumerate(vector1)]
def run_test(message, actual, expected):
if (actual != expected):
print(message)
def play_level(level):
if not level:
raise Exception("Please supply a valid Sokoban board.")
sok = Sokoboard(level)
message = ""
while not sok.won():
step = next_step(sok, message)
message = ""
if step == "Q": # quit game
print("You lose!")
return
elif step == "R": # restart level
sok = Sokoboard(level)
else:
if sok.is_valid_move(step):
sok = sok.move(step)
else:
message = "That is not a valid move"
os.system('cls' if os.name == 'nt' else 'clear')
print(sok.to_string())
print("You won!!!")
def main(level = ""):
play_level(level)
def test_board():
testlevel = """#####
#@$.#
#####"""
print("We're going to try this level:")
print(testlevel)
sok = Sokoboard(testlevel)
print("board \n", sok.board)
print("player at ", sok.player)
run_test("Should be able to move east into box with goal on other side", sok.is_valid_move("e"), True)
run_test("Should not be able to move west into wall", sok.is_valid_move("w"), False)
run_test("Should not be able to move south into wall", sok.is_valid_move("s"), False)
run_test("This game is not yet won", sok.won(), False)
testlevel1_1 = """#####
# @*#
#####"""
sok1_1 = sok.move("e")
run_test("After the move 'e', board should be\n" + testlevel1_1, sok1_1.to_string(), testlevel1_1)
testlevel2 = """#####
#.$@#
#.$ #
#####"""
sok2 = Sokoboard(testlevel2)
run_test("Should not be able to move East into wall", sok2.is_valid_move("e"), False)
run_test("Should be able to move West into box with goal on other side", sok2.is_valid_move("w"), True)
run_test("Should not be able to move north into wall", sok2.is_valid_move("n"), False)
run_test("Should be able to move south into empty space", sok2.is_valid_move("s"), True)
testlevel3 = """#####
#.. #
#$$@#
# #
#####"""
sok3 = Sokoboard(testlevel3)
run_test("Should not be able to move west into box when box on other side", sok3.is_valid_move("w"), False)
testlevel4 = """####
#@$#
####"""
sok4 = Sokoboard(testlevel4)
run_test("Should not be able to move east into box when wall on other side", sok4.is_valid_move("e"), False)
testlevel5 = """####
#@*#
####"""
sok5 = Sokoboard(testlevel5)
run_test("Game is won when all boxes are in goal squares", sok5.won(), True)
testlevel6_0 = """######
#. $@#
######"""
sok6 = Sokoboard(testlevel6_0)
sok6_1 = sok6.move("w")
run_test("If player moves off empty square, square becomes empty", sok6_1.get_square_at_location([1,4]), " ")
run_test("If player moves off empty square, player moves", sok6_1.get_square_at_location([1,3]), "@")
run_test("If player pushes box into empty square, box moves into empty square", sok6_1.get_square_at_location([1,2]), "$")
testlevel7 = """######
#@.$ #
# #
######"""
sok7 = Sokoboard(testlevel7)
sok7_1 = sok7.move("e")
run_test("If player moves onto goal, goal is still there underneath", sok7_1.get_square_at_location([1,2]), "+")
testlevel8 = """######
# +$ #
# #
######"""
sok8 = Sokoboard(testlevel8)
sok8_1 = sok8.move("s")
run_test("If player moves off of goal, goal is still in previous space", sok8_1.get_square_at_location([1,2]),".")
def test_quit():
testlevel8 = """######
# +$ #
# #
######"""
# Quit input
moves = ["s","Q"]
next_step = MagicMock(side_effect=moves)
game1 = play_level(testlevel8)
assert(True), "Did not run forever."
#Test restart ...
#Add arrow keys/WASD
if __name__ == '__main__':
minicosmos01 = """ #####
### #
# $ # ##
# # . #
# # #
## # #
#@ ###
##### """
minicosmos02 = """ #####
### #
# $ # ##
# # . #
# # #
##$#. #
#@ ###
##### """
levels = [minicosmos01, minicosmos02]
for level in levels:
main(level) | 478cb9990d21456af6eab16a63486f84229b37a5 | [
"Python"
] | 1 | Python | cperera/sokoban | 4bc20e23b38aa135b3c9aeb15b44707dbf36c53c | 0370c0c13b2b09f3ec2b0166671cc9af155210ff | |
refs/heads/master | <file_sep>import React from 'react';
import MyEditor from '../components/Editor';
import axios from 'axios';
import Alert from 'react-s-alert';
import { Link } from 'react-router-dom';
import { Editor, EditorState, convertToRaw, convertFromRaw } from 'draft-js';
import 'react-s-alert/dist/s-alert-default.css';
import 'react-s-alert/dist/s-alert-css-effects/flip.css';
class DocumentPage extends React.Component {
constructor(props) {
super(props);
this.state = {
title: 'Loading...',
}
}
componentWillMount() {
axios.get('https://morning-badlands-13664.herokuapp.com/document/' + this.props.match.params.id)
.then(resp => {
console.log(this.props.match.params.id);
this.setState({
title: resp.data.title
});
})
.catch(err => {
console.log("ERROR: Cannot retrieve document using axios request ", err);
});
}
saveDocument(newState) {
axios.post('https://morning-badlands-13664.herokuapp.com/saveDocument', {
text: newState,
id: this.props.match.params.id,
newRevision: {
revision: JSON.stringify(newState),
date: new Date()
}
})
.then(resp => {
if (resp.status === 200) {
console.log('success');
Alert.info("document has been saved!", {
position: 'top',
effect: 'flip',
timeout: 10000,
offset: 100
})
}
})
.catch(err => {
console.log("ERROR: Cannot retrieve document using axios request ", err);
});
}
autoSaveDocument(newState) {
axios.post('https://morning-badlands-13664.herokuapp.com/saveDocument', {
text: newState,
id: this.props.match.params.id
})
.then(resp => {
if (resp.status === 200) {
console.log('success');
}
})
.catch(err => {
console.log("ERROR: Cannot retrieve document using axios request ", err);
});
}
render() {
return (
<div className="editor-page">
<MyEditor
id={this.props.match.params.id}
editorState={this.state.editorState}
saveDocument={this.saveDocument.bind(this)}
autoSaveDocument={this.autoSaveDocument.bind(this)}
history={this.props.history}
documentReturnHandler={() => this.props.history.push('/documentlist')}
documentTitle={this.state.title}
documentId={this.props.match.params.id}
/>
<Alert stack={{limit: 1}} />
</div>
)
}
}
export default DocumentPage;
<file_sep># NodeBook
A collaborative text editor with easy to edit and share documents.
**Created July, 2017**
## Table of Contents
- [About](#about)
- [Installation Instructions](#installation-instructions)
- [Built With](#built-with)
- [Features](#features)
## Team
- [<NAME>](https://github.com/carokun)
- [<NAME>](https://github.com/teresaliu20)
- [<NAME>](https://github.com/sophiagrace)
- [<NAME>](https://github.com/crestwood204)
## About
NodeBook is a desktop app that allows for sharing documents and live collaboration. The text editor has numerous styles and fonts built in as well as search capabilities and access to revision history.
## Installation Instructions:
#### Prerequisites:
Requires Node.js
1. Download the repository
2. Run ```npm install``` to download the node modules
3. Run ```npm run webpack``` to bundle the code
4. Run ```npm run server``` to run the server
5. Run ```npm start``` to launch a new window
- You can launch multiple windows to test the collaborative functionality
## Built With
- Electron
- Frontend
- React.js
- Draft.js
- LESS
- HTML/CSS
- Backend
- Socket.io
- Express
- MongoDB
## Features
### Login and Register Page
When you first open the app you will be prompted with a login screen. For first time users, navigate to the register page and input your creditials before logging in!
<img width="1276" alt="screen shot 2017-07-28 at 12 09 13 pm" src="https://user-images.githubusercontent.com/23001355/28732706-b91898ba-738d-11e7-82bf-21e3b97c549b.png">
### Documents List
Your account will open to a preview of all your existing documents which are stored in MongoDB between sessions.
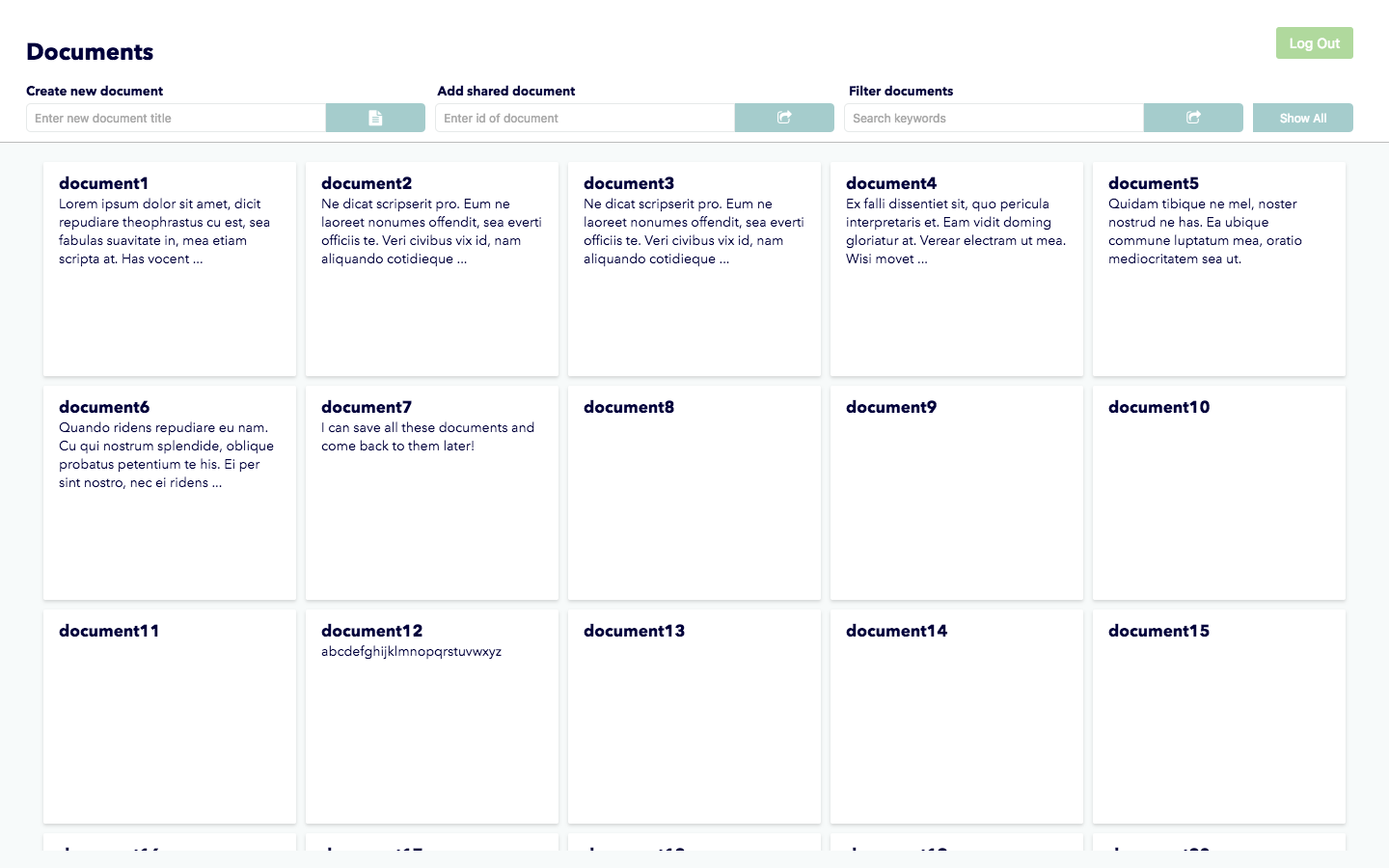
To search through the documents by title or content, type into the search bar on the upper right hand corner.
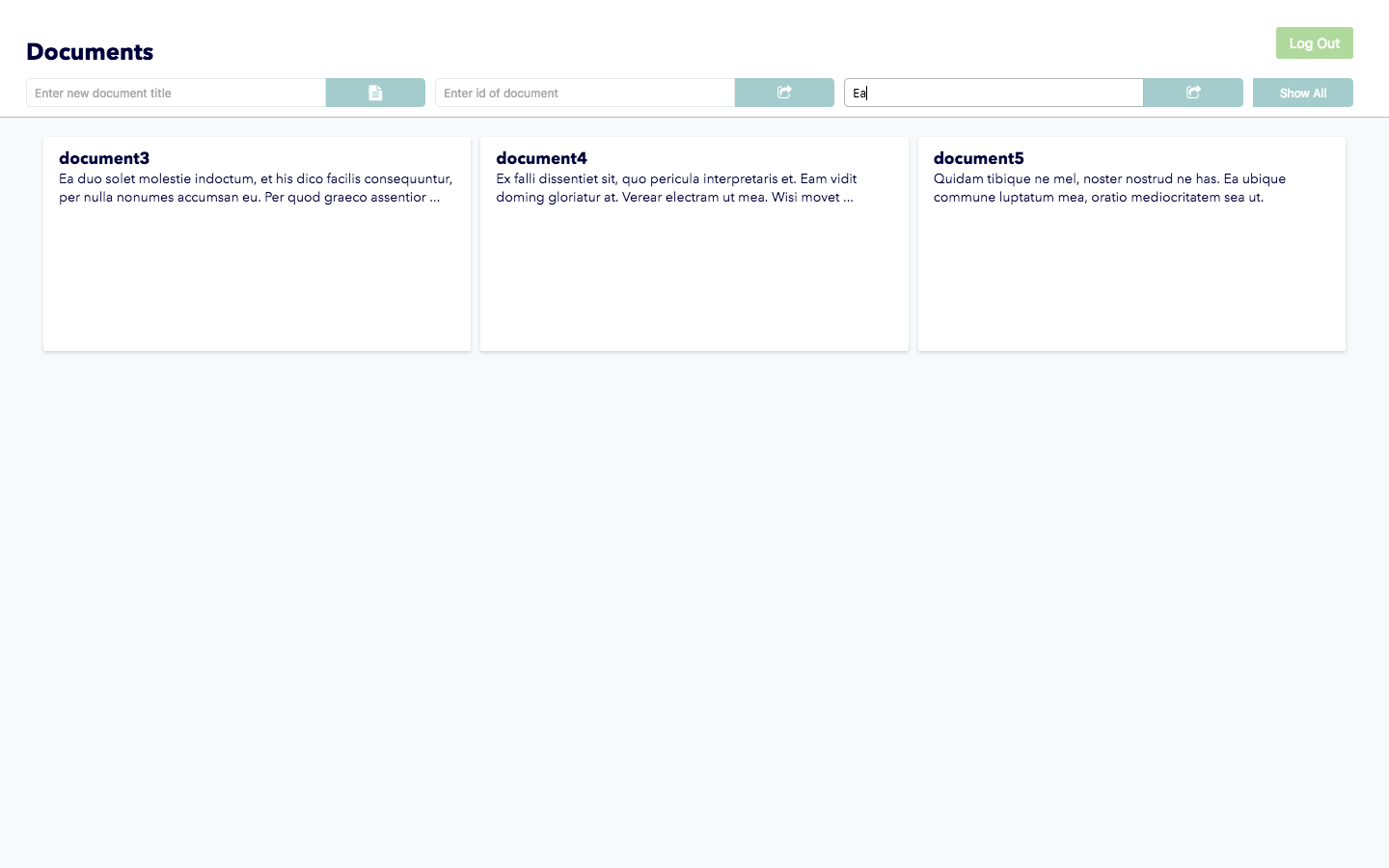
To navigate to a specific document, simply click the document's preview.
### Document View
We built our editor component on top of the Draft.js library. The document editor allows for typing and text decoration, including font size, color, and alignment, all of which are accessible from the top toolbar. There are keyboard shortcuts built in for common commands such as bolding (⌘B), italicizing (⌘I), and saving the document (⌘S). Some of the available effects are demoed in the picture below. The document will also autosave every 30 seconds so users don't lose their work!
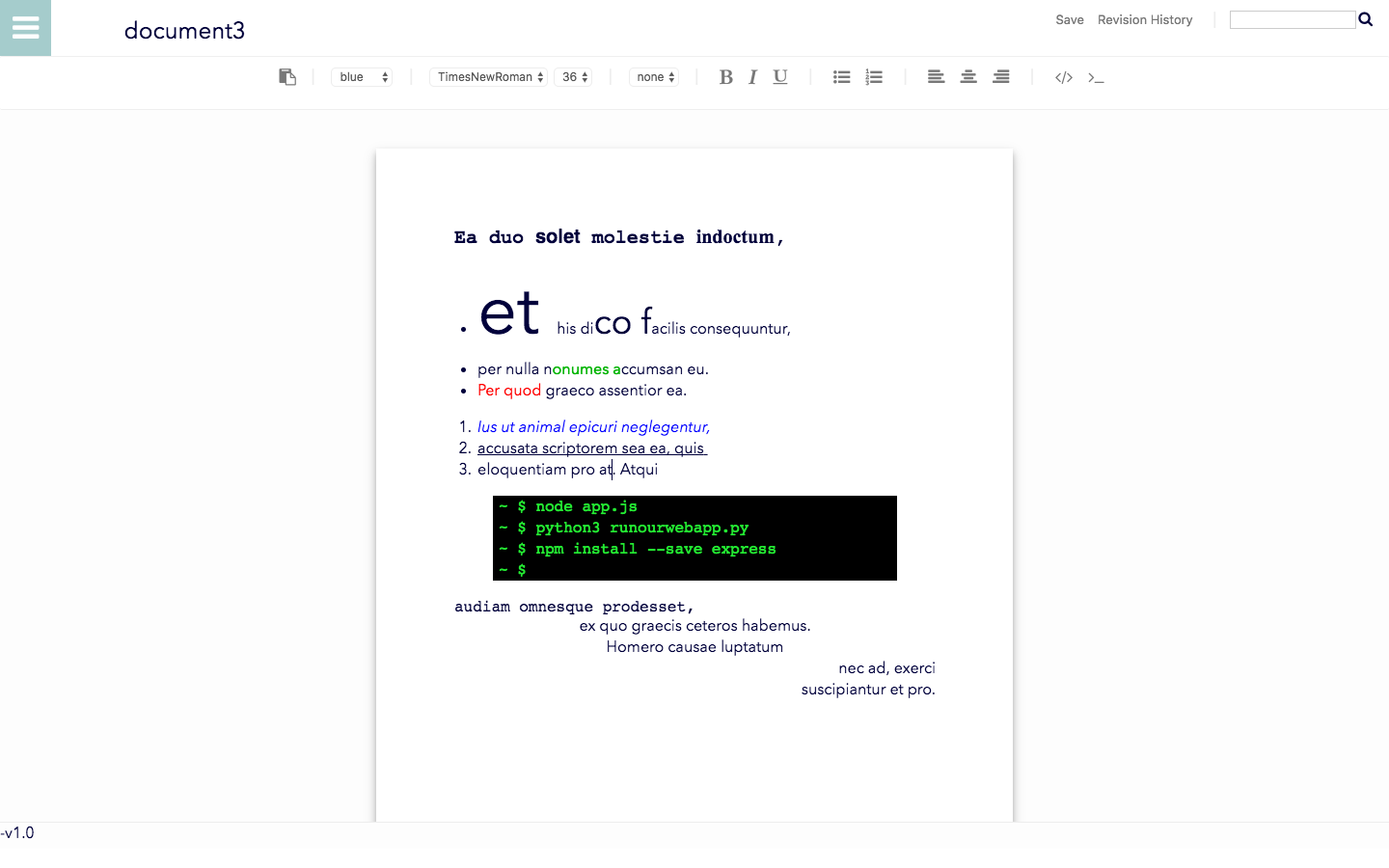
There is a search bar on the top right of the screen that allows users to search through their documents, highlighting the matching words and phrases.
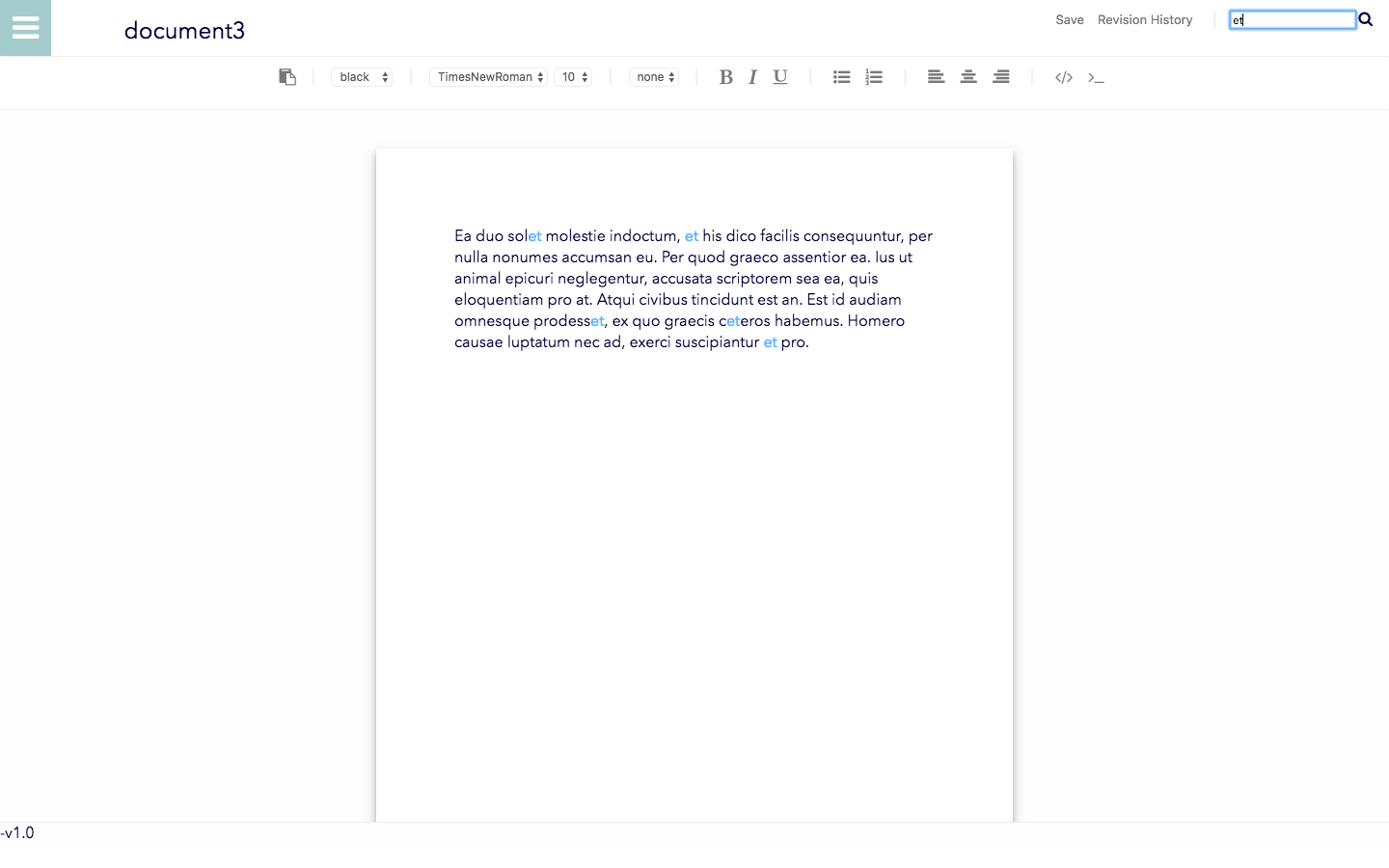
We also implemented live collaboration for shared documents using sockets. A preview of the collaborative features is shown below, to see more navigate to [Installation Instructions](#installation-instructions) and download the repo!
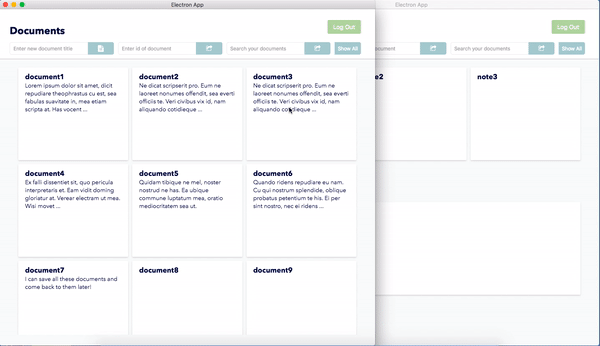
### Revision History
To see all of your past versions of a given document, navigate to the revision history page. There will be list of every saved version, sorted by date, on the right side of the screen. Click on one of the dates to see the old state of your document as well as add and remove differences between your old and current document.
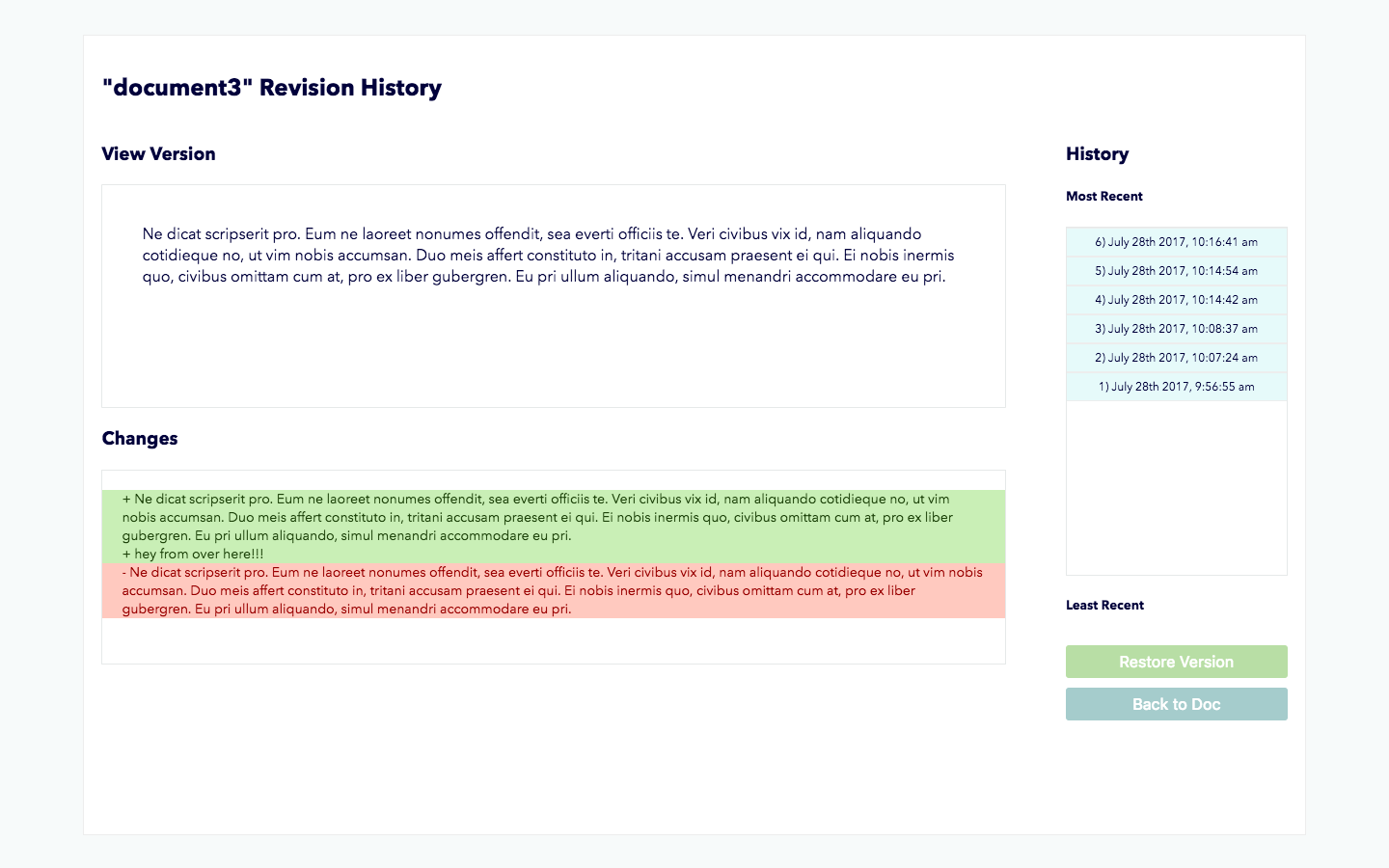
| a801c4be6f8dd30959a5797154b43f89212c8a3b | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | carokun/collaborative-text-editor | be2e12c1a7f2fa018b353ca2fe071511a533cf8a | 185f11496c780ca5b3f120de336b69797daa62db | |
refs/heads/master | <file_sep>import os
from global_settings import PROJECT_PATH, LOGGING
# Uncomment to put the application in non-debug mode. This is useful
# for testing error handling and messages.
# DEBUG = False
# TEMPLATE_DEBUG = DEBUG
# Override this to match the application endpoint
# FORCE_SCRIPT_NAME = ''
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': os.path.join(PROJECT_PATH, 'fabrydb.db')
}
}
# Non-restricted email port for development, run in a terminal:
# python -m smtpd -n -c DebuggingServer localhost:1025
EMAIL_PORT = 1025
EMAIL_SUBJECT_PREFIX = '[fabrydb] '
# This is used as a "seed" for various hashing algorithms. This must be set to
# a very long random string (40+ characters)
SECRET_KEY = 'abc123'
# Uncomment for additional logging. If using the 'rotating_file' handler
# you must create the `logs` directory in the project root.
# LOGGING['handlers'].update({
# 'stdout': {
# 'class': 'logging.StreamHandler',
# 'level': 'DEBUG',
# },
# 'rotating_file': {
# 'class': 'logging.handlers.RotatingFileHandler',
# 'level': 'DEBUG',
# 'filename': os.path.join(PROJECT_PATH, 'logs/debug.log')
# 'maxBytes': 2048,
# 'backupCount': 5,
# },
# })
#
# LOGGING['loggers'].update({
# 'django.db.backends': {
# 'handlers': ['rotating_file'],
# 'propagate': True,
# 'level': 'DEBUG',
# }
# })
<file_sep>import os
from global_settings import *
try:
from local_settings import *
from local_settings_secret import *
except ImportError:
import warnings
warnings.warn('Local settings have not been found (src.conf.local_settings). Trying to import Heroku config...')
try:
from local_settings_heroku import *
from local_settings_heroku_secret import *
warnings.warn('Local Heroku config loaded')
except ImportError:
warnings.warn('Heroku local settings not found neither (src.conf.local_settings_heroku)')
# FORCE_SCRIPT_NAME overrides the interpreted 'SCRIPT_NAME' provided by the
# web server. since the URLs below are used for various purposes outside of
# the WSGI application (static and media files), these need to be updated to
# reflect this alteration
if FORCE_SCRIPT_NAME:
ADMIN_MEDIA_PREFIX = os.path.join(FORCE_SCRIPT_NAME, ADMIN_MEDIA_PREFIX[1:])
STATIC_URL = os.path.join(FORCE_SCRIPT_NAME, STATIC_URL[1:])
MEDIA_URL = os.path.join(FORCE_SCRIPT_NAME, MEDIA_URL[1:])
LOGIN_URL = os.path.join(FORCE_SCRIPT_NAME, LOGIN_URL[1:])
LOGOUT_URL = os.path.join(FORCE_SCRIPT_NAME, LOGOUT_URL[1:])
LOGIN_REDIRECT_URL = os.path.join(FORCE_SCRIPT_NAME, LOGIN_REDIRECT_URL[1:])
# This is used as a "seed" for various hashing algorithms. This must be set to
# a very long random string (40+ characters)
SECRET_KEY = 'read from secret settings'
# Allow all host headers
ALLOWED_HOSTS = ['*']
# Static asset configuration
BASE_DIR = os.path.dirname(os.path.abspath(__file__))
STATIC_ROOT = os.path.join(PROJECT_PATH, '_site/static')
STATIC_URL = '/static/'
STATICFILES_DIRS = (
os.path.join(BASE_DIR, 'static'),
)
<file_sep>from django.shortcuts import render
from django.contrib.auth.decorators import login_required
from fabrydb.models import Dadosgerais
@login_required
def home(request):
n = Dadosgerais.objects.count()
extra_context = {}
extra_context['nb_patients'] = n
return render(request, 'site/home_box.html', extra_context)
<file_sep>[program:fabrydb-local]
directory = /home/devuser/.virtualenvs/fabrydb-env/fabrydb
command = /home/devuser/.virtualenvs/fabrydb-env/bin/uwsgi --ini /home/devuser/.virtualenvs/fabrydb-env/fabrydb/server/uwsgi/local.ini
autostart = true
autorestart = true
<file_sep>Django==1.5.11
South==0.8.1
Whoosh==2.4.0
avocado==2.3.10
django-guardian==1.2.4
django-haystack==2.0.0
django-preserialize==1.0.7
jdcal==1.0
jsonfield==0.9.4
modeltree==1.1.8
openpyxl==2.1.1
psycopg2==2.5.4
restlib2==0.4.3
serrano==2.3.10
six==1.8.0
wsgiref==0.1.2
<file_sep>[program:fabrydb-production]
directory = /home/devuser/webapps/fabrydb-env/fabrydb
command = /home/devuser/webapps/fabrydb-env/bin/uwsgi --ini /home/devuser/webapps/fabrydb-env/fabrydb/server/uwsgi/production.ini
autostart = true
autorestart = true
<file_sep>/etc/init.d/development.ini<file_sep>import os
# from global_settings import PROJECT_PATH
# Uncomment to put the application in non-debug mode. This is useful
# for testing error handling and messages.
DEBUG = False
TEMPLATE_DEBUG = DEBUG
# Override this to match the application endpoint
FORCE_SCRIPT_NAME = ''
# Non-restricted email port for development, run in a terminal:
# python -m smtpd -n -c DebuggingServer localhost:1025
EMAIL_PORT = 1025
EMAIL_SUBJECT_PREFIX = '[fabrydb Local] '
# Parse database configuration from $DATABASE_URL
import dj_database_url
DATABASES = {}
DATABASES['default'] = dj_database_url.config()
# Honor the 'X-Forwarded-Proto' header for request.is_secure()
SECURE_PROXY_SSL_HEADER = ('HTTP_X_FORWARDED_PROTO', 'https')
# Allow all host headers
ALLOWED_HOSTS = ['*']
# Static asset configuration
BASE_DIR = os.path.dirname(os.path.abspath(__file__))
STATIC_ROOT = '/app/_site/static'
STATIC_URL = '/static/'
STATICFILES_DIRS = (
os.path.join(BASE_DIR, 'static'),
)
# Simplified static file serving.
# https://warehouse.python.org/project/whitenoise/
# STATICFILES_STORAGE = 'whitenoise.django.GzipManifestStaticFilesStorage'
# Staticfiles_STORAGE = 'django.contrib.staticfiles.storage.CachedStaticFilesStorage'
# This is used as a "seed" for various hashing algorithms. This must be set to
# a very long random string (40+ characters)
SECRET_KEY = 'secret'
<file_sep>Django==1.5.11
South==0.8.1
Whoosh==2.4.0
avocado==2.3.10
dj-database-url==0.3.0
django-guardian==1.2.4
django-haystack==2.0.0
django-preserialize==1.0.7
gunicorn==19.1.1
jdcal==1.0
jsonfield==0.9.4
modeltree==1.1.8
openpyxl==2.1.1
psycopg2==2.5.4
restlib2==0.3.9
serrano>=2.3.0,<2.4.0
six==1.8.0
whitenoise==1.0.5
wsgiref==0.1.2
<file_sep>from django.contrib import admin
from fabrydb.models import (AvaliacaoCardiologica, AvaliacaoNeurologica,
AvaliacaoOftalmologica, AvaliacaoRenal, Banco,
BioquimicaGeral, DadosDaInfusao, Dadosgerais,
MssiCardiologico, MssiGeral,
MssiNeurologicoPsiquiatrico, MssiRenal,
Questionarios)
from fabrydb.models import (Tiposecg, TiposecocardiogramaI, Tiposholter2H,
Tiposacroparestesia,
TiposangioressonanciaMagnetica,
Tiposcerebrovascular,
Tiposevidenciadedisfuncaorenal, Tiposgenotipagem,
TiposmedicacoesEmUso,
Tiposmodificacoesnaespessuramiocardio,
TiposreacaoPosInfusao,
TiposcondutaAReacaoPosInfusao,
TiposresultadoDaBiopsia, Tipossexo, Tiposvertigem)
# Custom admin console
class FabrydbAdminSite(admin.AdminSite):
site_header = 'FaBRyDB'
fadmin = FabrydbAdminSite(name='fadmin')
# Registering models
class ListEcgInline(admin.TabularInline):
model = AvaliacaoCardiologica.category_ecg.through
class ListEcocardiogramaiInline(admin.TabularInline):
model = AvaliacaoCardiologica.category_ecocardiogramai.through
class ListHolter2hInline(admin.TabularInline):
model = AvaliacaoCardiologica.category_holter2h.through
class ListDadosgeraisInline(admin.TabularInline):
model = Dadosgerais.category_medicacoes_em_uso.through
class ListAvaliacaorenalInline(admin.TabularInline):
model = AvaliacaoRenal.category_resultados_da_biopsia.through
class AvaliacaoCardiologicaAdmin(admin.ModelAdmin):
list_display = ['id', 'data']
list_filter = ['category_ecg', 'id']
search_fields = ['id__nome']
inlines = [ListEcgInline, ListEcocardiogramaiInline, ListHolter2hInline, ]
class DadosgeraisAdmin(admin.ModelAdmin):
list_display = ['nome', 'data_de_nascimento', 'category_genotipagem']
list_filter = ['sexo', 'etnia', 'category_genotipagem', 'nome']
search_fields = ['nome']
inlines = [ListDadosgeraisInline, ]
class DadosDaInfusaoAdmin(admin.ModelAdmin):
list_display = ['id', 'data', 'category_reacao_pos_infusao',
'category_conduta_a_reacao_pos_infusao']
list_filter = ['data', 'category_conduta_a_reacao_pos_infusao__descricao',
'category_reacao_pos_infusao__descricao', 'id']
search_fields = ['id__nome']
change_form_template = 'admin/change_computed_fields.html'
def change_view(self, request, object_id, form_url='', extra_context=None):
"""Includes computed fields."""
d = DadosDaInfusao.objects.get(pk = object_id)
extra_context = extra_context or {}
extra_context['infusao'] = d
return super(DadosDaInfusaoAdmin, self).change_view(request, object_id,
form_url, extra_context=extra_context)
class AvaliacaoRenalAdmin(admin.ModelAdmin):
list_display = ['id', 'data', 'biopsia_renal']
list_filter = ['biopsia_renal', 'id']
search_fields = ['id__nome']
inlines = [ListAvaliacaorenalInline, ]
class MssiCardiologicoAdmin(admin.ModelAdmin):
list_display = ['id', 'data', 'modificacoes_na_espessura_do_miocardio']
list_filter = ['modificacoes_na_espessura_do_miocardio',
'anormalidades_no_ecg', 'marcapasso', 'hipertensao', 'id']
search_fields = ['id__nome']
class MssiRenalAdmin(admin.ModelAdmin):
list_display = ['id', 'data', 'evidencia_de_disfuncao_renal']
list_filter = ['evidencia_de_disfuncao_renal', 'id']
search_fields = ['id__nome']
class AvaliacaoNeurologicaAdmin(admin.ModelAdmin):
list_display = ['id', 'data', 'category_angioressonancia_magnetica']
list_filter = ['category_angioressonancia_magnetica', 'id']
search_fields = ['id__nome']
class AvaliacaoOftalmologicaAdmin(admin.ModelAdmin):
list_display = ['id', 'data']
list_filter = ['cornea_verticilata', 'tortuosidade_dos_vasos_retinianos',
'tortuosidade_dos_vasos_conjuntivais',
'opacidade_da_cornea', 'id']
search_fields = ['id__nome']
class BioquimicaGeralAdmin(admin.ModelAdmin):
list_display = ['id', 'data']
list_filter = ['id']
search_fields = ['id__nome']
class MssiGeralAdmin(admin.ModelAdmin):
list_display = ['id', 'data']
list_filter = ['aparencia_facial_caracteristica', 'angioqueratoma',
'edema', 'musculoesqueletico', 'cornea_verticilata',
'diaforese', 'dor_abdominal', 'diarreiaconstipacao',
'hemorroidas', 'pulmonar', 'nyha', 'id']
search_fields = ['id__nome']
class MssiNeurologicoPsiquiatricoAdmin(admin.ModelAdmin):
list_display = ['id', 'data']
list_filter = ['vertigem', 'acroparestesia', 'crises_de_febre_e_dor',
'cerebrovascular', 'depressao', 'fadiga', 'hipoatividade',
'id']
search_fields = ['id__nome']
fadmin.register(AvaliacaoCardiologica, AvaliacaoCardiologicaAdmin)
fadmin.register(AvaliacaoNeurologica, AvaliacaoNeurologicaAdmin)
fadmin.register(AvaliacaoOftalmologica, AvaliacaoOftalmologicaAdmin)
fadmin.register(AvaliacaoRenal, AvaliacaoRenalAdmin)
fadmin.register(Banco)
fadmin.register(BioquimicaGeral, BioquimicaGeralAdmin)
fadmin.register(DadosDaInfusao, DadosDaInfusaoAdmin)
fadmin.register(Dadosgerais, DadosgeraisAdmin)
fadmin.register(MssiCardiologico, MssiCardiologicoAdmin)
fadmin.register(MssiGeral, MssiGeralAdmin)
fadmin.register(MssiNeurologicoPsiquiatrico,
MssiNeurologicoPsiquiatricoAdmin)
fadmin.register(MssiRenal, MssiRenalAdmin)
fadmin.register(Questionarios)
fadmin.register(Tiposecg)
fadmin.register(TiposecocardiogramaI)
fadmin.register(Tiposholter2H)
fadmin.register(Tiposacroparestesia)
fadmin.register(TiposangioressonanciaMagnetica)
fadmin.register(Tiposcerebrovascular)
fadmin.register(Tiposevidenciadedisfuncaorenal)
fadmin.register(Tiposgenotipagem)
fadmin.register(TiposmedicacoesEmUso)
fadmin.register(Tiposmodificacoesnaespessuramiocardio)
fadmin.register(TiposreacaoPosInfusao)
fadmin.register(TiposcondutaAReacaoPosInfusao)
fadmin.register(TiposresultadoDaBiopsia)
fadmin.register(Tipossexo)
fadmin.register(Tiposvertigem)
admin.site.register(AvaliacaoCardiologica, AvaliacaoCardiologicaAdmin)
admin.site.register(AvaliacaoNeurologica, AvaliacaoNeurologicaAdmin)
admin.site.register(AvaliacaoOftalmologica, AvaliacaoOftalmologicaAdmin)
admin.site.register(AvaliacaoRenal, AvaliacaoRenalAdmin)
admin.site.register(Banco)
admin.site.register(BioquimicaGeral, BioquimicaGeralAdmin)
admin.site.register(DadosDaInfusao, DadosDaInfusaoAdmin)
admin.site.register(Dadosgerais, DadosgeraisAdmin)
admin.site.register(MssiCardiologico, MssiCardiologicoAdmin)
admin.site.register(MssiGeral, MssiGeralAdmin)
admin.site.register(MssiNeurologicoPsiquiatrico,
MssiNeurologicoPsiquiatricoAdmin)
admin.site.register(MssiRenal, MssiRenalAdmin)
admin.site.register(Questionarios)
admin.site.register(Tiposecg)
admin.site.register(TiposecocardiogramaI)
admin.site.register(Tiposholter2H)
admin.site.register(Tiposacroparestesia)
admin.site.register(TiposangioressonanciaMagnetica)
admin.site.register(Tiposcerebrovascular)
admin.site.register(Tiposevidenciadedisfuncaorenal)
admin.site.register(Tiposgenotipagem)
admin.site.register(TiposmedicacoesEmUso)
admin.site.register(Tiposmodificacoesnaespessuramiocardio)
admin.site.register(TiposreacaoPosInfusao)
admin.site.register(TiposcondutaAReacaoPosInfusao)
admin.site.register(TiposresultadoDaBiopsia)
admin.site.register(Tipossexo)
admin.site.register(Tiposvertigem)
<file_sep>from django import template
from django.conf import settings
register = template.Library()
@register.inclusion_tag('site/mailto_support.html')
def mailto_support():
return {"support_email": settings.SUPPORT_EMAIL }
| 5a84a343a8b2f37be50af2543991e1f29de5965f | [
"Python",
"Text",
"INI"
] | 11 | Python | glabilloy/fabrydb | c4880eee70378c3d4fb9d334a41d7e2b6c7a4f3a | bc1da1046b7849052c78133835dfa79ca98eeabf | |
refs/heads/master | <repo_name>jxunix/utility<file_sep>/Makefile
# Makefile
.PHONY: pull push config install
ROOT = $(shell dirname $(abspath $(lastword $(MAKEFILE_LIST))))
all: push
pull:
git pull origin master
push:
git add -A
git commit -m "update"
git push origin master
config: pull
ln -sf ${ROOT}/.bash_aliases ~/.bash_aliases
ln -sf ${ROOT}/.vimrc ~/.vimrc
ln -sf ${ROOT}/.gitconfig ~/.gitconfig
ln -sfn ${ROOT}/ftplugin ~/.vim/ftplugin
ln -sfn ${ROOT}/colors ~/.vim/colors
install:
./install.sh
<file_sep>/README.md
# utility
1. Set up `Vundle` </br>
$ git clone https://github.com/gmarik/Vundle.vim.git ~/.vim/bundle/Vundle.vim
2. Install plugin `YouCompleteMe` </br>
$ git clone https://github.com/Valloric/YouCompleteMe ~/.vim/bundle/YouCompleteMe </br>
$ cd ~/.vim/bundle/YouCompleteMe </br>
$ ./install.py --clang-completer </br>
For more information, please visit https://github.com/valloric/youcompleteme.
3. Install plugins </br>
Launch vim and run :PluginInstall
<file_sep>/install.sh
#!/bin/bash
sudo -i
apt install -y build-essential
apt install -y cmake
apt install -y ctags
apt install -y default-jdk
apt install -y git
apt install -y python3-dev
apt update -y
apt dist-upgrade -y
exit
<file_sep>/.bash_aliases
#----------------------------------------------------------------------
# Renaming
#----------------------------------------------------------------------
alias ls="ls --color=always"
alias grep="grep --color=always"
alias vi="vim"
#----------------------------------------------------------------------
# Variables
#----------------------------------------------------------------------
export vimrc=~/.vimrc
export profile=~/.bash_aliases
#----------------------------------------------------------------------
# Functions
#----------------------------------------------------------------------
v_aux() {
if [ "${1: -1}" == "." ]; then
vim -O $1{h,cpp}
elif [ "${1: -4}" == ".cpp" ]; then
vim "$1"
elif [ "${1: -2}" == ".h" ]; then
vim "$1"
else
vim -O $1.{h,cpp}
fi
}
cd_aux() {
if [ -d ../"$1" ]; then
cd ../"$1"
else
cd "$1"
fi
}
parse_git_branch() {
git branch 2> /dev/null | sed -e '/^[^*]/d' -e 's/* \(.*\)/ (\1)/'
}
#----------------------------------------------------------------------
# Alias
#----------------------------------------------------------------------
for dir in "$HOME"/*; do
[ -d "$dir" ] && alias $(basename "$dir")="cd $dir"
done
for dir in "$HOME"/*; do
[ -d "$dir" ] && export $(basename "$dir")="$dir"
done
alias src="cd_aux src"
alias tst="cd_aux test"
alias build="cd_aux build"
alias ll="ls -l"
alias lla="ll -A"
alias llt="ll -t"
alias v="v_aux"
alias sp="source $profile"
alias vp="vim $profile"
alias vv="vim $vimrc"
alias gs="clear; grep --include=\*.{cpp,h,cc,hh} --exclude=\*.t.{h,cpp} -nr"
alias f="find * -name"
alias tag="ctags -R *"
alias update="sudo apt update -y; sudo apt dist-upgrade -y"
alias nb='jupyter notebook'
#----------------------------------------------------------------------
# Settings
#----------------------------------------------------------------------
set -o vi
| 74b3bff7298979ca60a9305cfef83421b6096604 | [
"Markdown",
"Makefile",
"Shell"
] | 4 | Makefile | jxunix/utility | 2e87d3d996290985372154c107e46478e1816ff5 | 4d3ddefe2a9df83c8d5dc3d889c2dc299e847895 | |
refs/heads/master | <file_sep>package com.cr_wd.jenkins.plugins.wordpressrepo;
import hudson.Extension;
import hudson.FilePath;
import hudson.Launcher;
import hudson.model.AbstractBuild;
import hudson.model.AbstractProject;
import hudson.model.BuildListener;
import hudson.tasks.BuildStepDescriptor;
import hudson.tasks.Builder;
import hudson.util.FormValidation;
import net.sf.json.JSONObject;
import org.kohsuke.stapler.DataBoundConstructor;
import org.kohsuke.stapler.QueryParameter;
import org.kohsuke.stapler.StaplerRequest;
import javax.servlet.ServletException;
import java.io.IOException;
public class WordpressPluginBuilder extends WordpressBuilder {
private final String name;
// Fields in config.jelly must match the parameter names in the "DataBoundConstructor"
@DataBoundConstructor
public WordpressPluginBuilder(String name) {
this.name = name;
}
/**
* We'll use this from the <tt>config.jelly</tt>.
*/
public String getName() {
return name;
}
@Override
public boolean perform(AbstractBuild build, Launcher launcher, BuildListener listener) {
return true;
}
// Overridden for better type safety.
// If your plugin doesn't really define any property on Descriptor,
// you don't have to do this.
@Override
public DescriptorImpl getDescriptor() {
return (DescriptorImpl) super.getDescriptor();
}
/**
* Descriptor for {@link WordpressBuilder}. Used as a singleton.
* The class is marked as public so that it can be accessed from views.
* <p/>
* <p/>
* See <tt>src/main/resources/hudson/plugins/hello_world/HelloWorldBuilder/*.jelly</tt>
* for the actual HTML fragment for the configuration screen.
*/
@Extension // This indicates to Jenkins that this is an implementation of an extension point.
public static final class DescriptorImpl extends BuildStepDescriptor<Builder> {
/**
* To persist global configuration information,
* simply store it in a field and call save().
* <p/>
* <p/>
* If you don't want fields to be persisted, use <tt>transient</tt>.
*/
private boolean useFrench;
/**
* Performs on-the-fly validation of the form field 'name'.
*
* @param value This parameter receives the value that the user has typed.
* @return Indicates the outcome of the validation. This is sent to the browser.
*/
public FormValidation doCheckName(@QueryParameter String value)
throws IOException, ServletException {
if (value.length() == 0)
return FormValidation.error("Please set a name");
if (value.length() < 4)
return FormValidation.warning("Isn't the name too short?");
return FormValidation.ok();
}
public boolean isApplicable(Class<? extends AbstractProject> aClass) {
// Indicates that this builder can be used with all kinds of project types
return true;
}
/**
* This human readable name is used in the configuration screen.
*/
public String getDisplayName() {
return "Build Wordpress Plugin";
}
@Override
public boolean configure(StaplerRequest req, JSONObject formData) throws FormException {
// To persist global configuration information,
// set that to properties and call save().
useFrench = formData.getBoolean("useFrench");
// ^Can also use req.bindJSON(this, formData);
// (easier when there are many fields; need set* methods for this, like setUseFrench)
save();
return super.configure(req, formData);
}
/**
* This method returns true if the global configuration says we should speak French.
* <p/>
* The method name is bit awkward because global.jelly calls this method to determine
* the initial state of the checkbox by the naming convention.
*/
public boolean getUseFrench() {
return useFrench;
}
}
}
<file_sep>package com.cr_wd.jenkins.plugins.wordpressrepo;
import hudson.tasks.Builder;
public abstract class WordpressBuilder extends Builder {
}
| f2a18f6388e1b2ecbfa55d0eb4a6a5864567d2da | [
"Java"
] | 2 | Java | croemmich/Jenkins-Wordpress-Builder | ec586c9e287c6ac94ad86a89c4b632600679e8c8 | fc62d7f6ef7d1183fae31f0f18c3b1a99959bc7f | |
refs/heads/master | <repo_name>manishyadav436/MyFirstRepo<file_sep>/vendor/ernysans/laraworld/tests/TimeZonesTest.php
<?php
use ErnySans\Laraworld\Models\TimeZones;
use Illuminate\Support\Facades\Artisan;
use Illuminate\Support\Facades\File;
use Illuminate\Support\Facades\Schema;
class TimeZonesTest extends AppTestCase
{
/**
* Check if JSON file exist
*/
public function testItFetchesJsonFile()
{
$collection = TimeZones::allJSON();
$this->assertNotNull($collection);
}
/**
* Publish migrations for testing
*/
public function testPublishedMigrations()
{
$source = __DIR__ . '/../src/Database/migrations/0000_00_00_000000_create_time_zones_table.php';
$destination = database_path('migrations/0000_00_00_000000_create_time_zones_table.php');
File::copy($source, $destination);
$success = File::exists($destination);
$this->assertTrue($success);
}
/**
* Publish seeds for testing
*/
public function testPublishedSeeds()
{
$source = __DIR__ . '/../src/Database/seeds/TimeZonesTableSeeder.php';
$destination = database_path('seeds/TimeZonesTableSeeder.php');
File::copy($source, $destination);
$success = File::exists($destination);
$this->assertTrue($success);
}
/**
* Run migrations
* @depends testPublishedMigrations
*/
public function testItHasMigrated()
{
Artisan::call('migrate');
$this->assertTrue(true);
}
/**
* Check if the table exist
* @depends testItHasMigrated
*/
public function testItCheckForTable()
{
$collection = Schema::hasTable('time_zones');
$this->assertTrue($collection);
}
/**
* Seed the table
* @depends testPublishedSeeds
* @depends testItFetchesJsonFile
*/
public function testItHasSeeded()
{
Artisan::call('db:seed');
$this->assertTrue(true);
}
/**
* Check if the table was seeded
* @depends testItHasSeeded
*/
public function testItCanSeed()
{
$collection = TimeZones::all();
$this->assertNotNull($collection->count());
}
/**
* Publish migrations for testing
* @depends testItHasMigrated
*/
public function testDeleteMigrations()
{
$base = database_path('migrations/****_**_**_******_create_time_zones_table.php');
$source = File::glob($base);
$route = $source[0];
File::delete($route);
$success = File::exists($route);
$this->assertFalse($success);
}
/**
* Publish seeds for testing
* @depends testItHasSeeded
*/
public function testDeleteSeeds()
{
$source = database_path('seeds/TimeZonesTableSeeder.php');
File::delete($source);
$success = File::exists($source);
$this->assertFalse($success);
}
}
<file_sep>/vendor/ernysans/laraworld/src/LaraworldServiceProvider.php
<?php
namespace ErnySans\Laraworld;
use ErnySans\Laraworld\Models\Countries;
use ErnySans\Laraworld\Models\Languages;
use ErnySans\Laraworld\Models\TimeZones;
use Illuminate\Support\ServiceProvider;
use Illuminate\Support\Facades\File;
class LaraworldServiceProvider extends ServiceProvider
{
/**
* Register bindings in the container.
*
* @return void
*/
public function register()
{
$this->app->bind('Countries', function() {
return new Countries;
});
$this->app->bind('Languages', function() {
return new Languages;
});
$this->app->bind('TimeZones', function() {
return new TimeZones;
});
}
/**
* Perform post-registration booting of services.
*
* @return void
*/
public function boot()
{
// Countries Migration =========================
$countries_migration = $this->getTimePath('create_countries_table', 'migrations');
$this->publishes([
__DIR__.'/Database/migrations/0000_00_00_000000_create_countries_table.php' => database_path($countries_migration)
], 'migrate-countries');
// Countries Seed ==============================
$this->publishes([
__DIR__.'/Database/seeds/CountriesTableSeeder.php' => database_path('seeds/CountriesTableSeeder.php')
], 'seed-countries');
// Languages Migration =========================
$languages_migration = $this->getTimePath('create_languages_table', 'migrations');
$this->publishes([
__DIR__.'/Database/migrations/0000_00_00_000000_create_languages_table.php' => database_path($languages_migration)
], 'migrate-languages');
// Languages Seed ==============================
$this->publishes([
__DIR__.'/Database/seeds/LanguagesTableSeeder.php' => database_path('seeds/LanguagesTableSeeder.php')
], 'seed-languages');
// Time Zones Migration ========================
$time_zones_migration = $this->getTimePath('create_time_zones_table', 'migrations');
$this->publishes([
__DIR__.'/Database/migrations/0000_00_00_000000_create_time_zones_table.php' => database_path($time_zones_migration)
], 'migrate-time-zone');
// Time Zones Seed =============================
$this->publishes([
__DIR__.'/Database/seeds/TimeZonesTableSeeder.php' => database_path('seeds/TimeZonesTableSeeder.php')
], 'seed-time-zone');
}
/**
* Get the date prefix for the migration.
*
* @return string
*/
protected function getDatePrefix()
{
return date('Y_m_d_His');
}
/**
* If the file exist retrieve the same name
*
* @param $name
* @return null
*/
private function getMigrationCurrentName($name) {
$base = database_path('migrations/****_**_**_******_'.$name.'.php');
$source = File::glob($base);
if ($source) {
return basename($source[0], ".php");
}
return null;
}
/**
* Get the full path name to the migration.
*
* @param string $name
* @param string $path
* @return string
*/
protected function getTimePath($name, $path)
{
if ($this->getMigrationCurrentName($name)) {
$txt = $this->getMigrationCurrentName($name);
}
else {
$txt = $this->getDatePrefix().'_'.$name;
}
return $path.'/'.$txt.'.php';
}
}<file_sep>/app/Http/Controllers/DataVoiceController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\DataVoice;
use Yajra\Datatables\Datatables;
use Validator;
use Rinvex\Country\Country;
class DataVoiceController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$empdata = DataVoice::all();
return view('emp.index', compact('empdata'));
}
public function getUsers(){
return Datatables::of(DataVoice::query())
->addColumn('action', function($row){
$btn = '<a href="javascript:void(0)" class="edit btn btn-primary btn-sm">View</a>';
return $btn;
})
->rawColumns(['action'])
->make(true);
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
return view('emp.create');
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$request->validate([
'emp_id'=>'required|numeric',
'emp_name'=>'required',
'country_code'=>'required',
'mobile'=>'required',
'email'=>'required',
'dob'=>'required',
'profile'=>'mimes:jpg,png|required|max:10000'
]);
$data = new DataVoice([
'emp_id' => $request->get('emp_id'),
'emp_name' => $request->get('emp_name'),
'country_code' => $request->get('country_code'),
'mobile' => $request->get('mobile'),
'email' => $request->get('email'),
'dob' => $request->get('dob'),
]);
$image = $request->file('profile');
$new_name = rand() . '.' . $image->getClientOriginalExtension();
$image->move(public_path('images'), $new_name);
$filename = $image;
$data->profile = $filename;
$data->save();
return redirect('/home')->with('success', 'Contact saved!');
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
$emp = DataVoice::find($id);
return view('emp.edit', compact('emp'));
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
$request->validate([
'emp_id'=>'required',
'emp_name'=>'required',
'country_code'=>'required',
'mobile'=>'required',
'email'=>'required',
'dob'=>'required',
'profile'=>'required'
]);
$data = DataVoice::find($id);
$data->emp_id = $request->get('emp_id');
$data->emp_name = $request->get('emp_name');
$data->country_code = $request->get('country_code');
$data->mobile = $request->get('mobile');
$data->email = $request->get('email');
$data->dob = $request->get('dob');
$data->profile = $request->get('profile');
$data->save();
return redirect('/home')->with('success', 'Data Updated!');
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
$empdata = DataVoice::find($id);
$empdata->delete();
return redirect('/home')->with('success', 'Data Deleted !');;
}
}
<file_sep>/vendor/ernysans/laraworld/src/Database/seeds/LanguagesTableSeeder.php
<?php
use Illuminate\Database\Seeder;
use ErnySans\Laraworld\Models\Languages;
class LanguagesTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
// Empty the table
Languages::truncate();
// Get all from the JSON file
$JSON_languages = Languages::allJSON();
foreach ($JSON_languages as $language) {
Languages::create([
'alpha3' => ((isset($language['alpha3'])) ? $language['alpha3'] : null),
'alpha2' => ((isset($language['alpha2'])) ? $language['alpha2'] : null),
'english' => ((isset($language['english'])) ? $language['english'] : null),
]);
}
}
}
<file_sep>/vendor/ernysans/laraworld/src/Facades/Languages.php
<?php
namespace ErnySans\Laraworld\Facades;
use Illuminate\Support\Facades\Facade;
class Languages extends Facade
{
protected static function getFacadeAccessor() {
return 'Languages';
}
}<file_sep>/vendor/ernysans/laraworld/src/Models/Languages.php
<?php
namespace ErnySans\Laraworld\Models;
use Illuminate\Database\Eloquent\Model;
class Languages extends Model
{
/**
* The database table used by the model.
*
* @var string
*/
protected $table = 'languages';
/**
* The attributes excluded from the model's JSON form.
*
* @var array
*/
protected $hidden = [
'id',
'created_at',
'updated_at'
];
/**
* Protect $dates
*
* @var array
*/
protected $dates = [
'created_at',
'updated_at'
];
/**
* he attributes that are mass assignable.
*
* @var array
*/
protected $fillable = [
'alpha3',
'alpha2',
'english',
];
/**
* Get all the countries from the JSON file.
*
* @return array
*/
public static function allJSON()
{
$route = dirname(dirname(__FILE__)) . '/Database/data/languages.json';
return json_decode(file_get_contents($route), true);
}
}
<file_sep>/vendor/ernysans/laraworld/tests/AppTestCase.php
<?php
use ErnySans\Laraworld\LaraworldServiceProvider;
use Illuminate\Support\Facades\Artisan;
use Illuminate\Foundation\Testing\WithoutMiddleware;
use Illuminate\Foundation\Testing\DatabaseMigrations;
use Illuminate\Console\Command;
use Symfony\Component\Console\Output\ConsoleOutputInterface;
class AppTestCase extends Illuminate\Foundation\Testing\TestCase
{
use WithoutMiddleware, DatabaseMigrations;
/**
* Creates the application.
*
* @return \Illuminate\Foundation\Application
*/
public function createApplication()
{
$app = require __DIR__.'/../vendor/laravel/laravel/bootstrap/app.php';
$app->make(Illuminate\Contracts\Console\Kernel::class)->bootstrap();
return $app;
}
/**
* Run before every test
*/
public function setUp()
{
parent::setUp();
}
/**
* Run after every test
*/
public function tearDown()
{
//Artisan::call('migrate:reset');
parent::tearDown();
}
}<file_sep>/vendor/ernysans/laraworld/README.md
[](https://travis-ci.org/ernysans/laraworld) [](https://packagist.org/packages/ernysans/laraworld) [](https://packagist.org/packages/ernysans/laraworld) [](https://packagist.org/packages/ernysans/laraworld) [](https://packagist.org/packages/ernysans/laraworld)
# Laraworld
Countries, Languages and Time Zones Package for Laravel and Lumen 5.*
Please check the [Known Issues](https://github.com/ernysans/laraworld/issues) section before reporting a new one.

## Installation
This package can be installed through Composer.
```bash
$ composer require ernysans/laraworld
```
When using Laravel there is a service provider that you can make use of.
```php
// app/config/app.php
'providers' => [
'...',
ErnySans\Laraworld\LaraworldServiceProvider::class,
];
```
Configure the aliases to make use of the Facades.
```php
// app/config/app.php
'aliases' => [
'...',
'Countries' => ErnySans\Laraworld\Facades\Countries::class,
'TimeZones' => ErnySans\Laraworld\Facades\TimeZones::class,
'Languages' => ErnySans\Laraworld\Facades\Languages::class,
];
```
> If you are using Laravel 5.5 it's not required to manually add the service provider and aliases.
Publish Migrations and Seeds.
```bash
$ php artisan vendor:publish --provider="ErnySans\Laraworld\LaraworldServiceProvider"
```
Prepare Seeds.
```php
// database/seeds/DatabaseSeeder.php
class DatabaseSeeder extends Seeder
{
public function run()
{
// Seed the countries
$this->call(CountriesTableSeeder::class);
// Seed the Time Zones
$this->call(TimeZonesTableSeeder::class);
// Seed the Languages
$this->call(LanguagesTableSeeder::class);
}
}
```
Run Migrations and Seed the tables
```bash
$ php artisan migrate
$ php artisan db:seed
```
## Use
These methods are provided:
### Collection Methods
When using Laravel you can work with the [Collection Available Methods](https://laravel.com/docs/master/collections).
### JSON file (Optional)
Use this methods If you want to get all the data from the JSON file:
* `Countries::allJSON()`: Get all the countries from the JSON file.
* `Languages::allJSON()`: Get all the languages from the JSON file.
* `TimeZones::allJSON()`: Get all the time zones from the JSON file.
## Contributing
Thank you for considering contributing to the Laraworld package!. Remember to run PHPUnit tests before sending a new pull request.
## License
This package is open-sourced licensed under the [MIT license](http://opensource.org/licenses/MIT).
<file_sep>/database/seeds/CountriesTableSeeder.php
<?php
use Illuminate\Database\Seeder;
use ErnySans\Laraworld\Models\Countries;
class CountriesTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
// Empty the table
Countries::truncate();
// Get all from the JSON file
$JSON_countries = Countries::allJSON();
foreach ($JSON_countries as $country) {
Countries::create([
'capital' => ((isset($country['capital'])) ? $country['capital'] : null),
'citizenship' => ((isset($country['citizenship'])) ? $country['citizenship'] : null),
'country_code' => ((isset($country['country_code'])) ? $country['country_code'] : null),
'currency' => ((isset($country['currency'])) ? $country['currency'] : null),
'currency_code' => ((isset($country['currency_code'])) ? $country['currency_code'] : null),
'currency_sub_unit' => ((isset($country['currency_sub_unit'])) ? $country['currency_sub_unit'] : null),
'full_name' => ((isset($country['full_name'])) ? $country['full_name'] : null),
'iso_3166_2' => ((isset($country['iso_3166_2'])) ? $country['iso_3166_2'] : null),
'iso_3166_3' => ((isset($country['iso_3166_3'])) ? $country['iso_3166_3'] : null),
'name' => ((isset($country['name'])) ? $country['name'] : null),
'region_code' => ((isset($country['region_code'])) ? $country['region_code'] : null),
'sub_region_code' => ((isset($country['sub_region_code'])) ? $country['sub_region_code'] : null),
'eea' => (bool)$country['eea'],
'calling_code' => ((isset($country['calling_code'])) ? $country['calling_code'] : null),
'currency_symbol' => ((isset($country['currency_symbol'])) ? $country['currency_symbol'] : null),
]);
}
}
}
<file_sep>/vendor/ernysans/laraworld/src/Facades/TimeZones.php
<?php
namespace ErnySans\Laraworld\Facades;
use Illuminate\Support\Facades\Facade;
class TimeZones extends Facade
{
protected static function getFacadeAccessor() {
return 'TimeZones';
}
}<file_sep>/vendor/ernysans/laraworld/src/Models/Countries.php
<?php
namespace ErnySans\Laraworld\Models;
use Illuminate\Database\Eloquent\Model;
class Countries extends Model
{
/**
* The database table used by the model.
*
* @var string
*/
protected $table = 'countries';
/**
* The attributes excluded from the model's JSON form.
*
* @var array
*/
protected $hidden = [
'id',
'created_at',
'updated_at'
];
/**
* Protect $dates
*
* @var array
*/
protected $dates = [
'created_at',
'updated_at'
];
/**
* he attributes that are mass assignable.
*
* @var array
*/
protected $fillable = [
'name',
'full_name',
'capital',
'citizenship',
'currency',
'currency_code',
'currency_sub_unit',
'currency_symbol',
'country_code',
'region_code',
'sub_region_code',
'eea',
'calling_code',
'iso_3166_2',
'iso_3166_3',
];
/**
* Get all the countries from the JSON file.
*
* @return array
*/
public static function allJSON()
{
$route = dirname(dirname(__FILE__)) . '/Database/data/countries.json';
return json_decode(file_get_contents($route), true);
}
}<file_sep>/database/seeds/DatabaseSeeder.php
<?php
use Illuminate\Database\Seeder;
class DatabaseSeeder extends Seeder
{
/**
* Seed the application's database.
*
* @return void
*/
public function run()
{
// Seed the countries
$this->call(CountriesTableSeeder::class);
// Seed the Time Zones
$this->call(TimeZonesTableSeeder::class);
// Seed the Languages
$this->call(LanguagesTableSeeder::class);
}
}
<file_sep>/app/DataVoice.php
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class DataVoice extends Model
{
protected $fillable = [
'emp_id', 'emp_name', 'country_code','mobile','email','dob','profile',
];
}
| 775ac7a4d7d35bafdb0d651fbf076043e1b19cf7 | [
"Markdown",
"PHP"
] | 13 | PHP | manishyadav436/MyFirstRepo | 41feb33f474ebcf0a03c53bb59a5930d8eb11d7d | a73ea63e839f3208623b0d1262856b32472d823d | |
refs/heads/master | <file_sep>import {Component, OnInit} from '@angular/core';
import * as cytoscape from 'cytoscape';
import {FormControl, FormGroup} from '@angular/forms';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent implements OnInit {
nodeAddForm = new FormGroup({
name: new FormControl(''),
});
edgeAddForm = new FormGroup({
source: new FormControl(''),
target: new FormControl(''),
});
dfsOutput: string[] = [];
stylesheet = [
{
selector: 'node',
style: {
content: 'data(id)'
}
},
{
selector: 'edge',
style: {
content: 'data(label)',
'curve-style': 'bezier',
'target-arrow-shape': 'triangle',
width: 4,
'line-color': '#ddd',
'target-arrow-color': '#ddd'
}
},
{
selector: '.highlighted',
style: {
'background-color': '#61bffc',
'line-color': '#61bffc',
'target-arrow-color': '#61bffc',
'transition-property': 'background-color, line-color, target-arrow-color',
'transition-duration': '0.5s'
}
},
];
cy: any;
dfs: any;
i: number;
ngOnInit(): void {
this.cy = cytoscape({
container: document.getElementById('cy'),
boxSelectionEnabled: false,
autounselectify: true,
style: this.stylesheet,
elements: {
nodes: [
{data: {id: 'a'}},
{data: {id: 'b'}},
],
edges: [
{data: {id: 'ab', weight: 1, source: 'a', target: 'b', label: 'ab'}},
]
},
layout: {
name: 'breadthfirst',
directed: true,
roots: '#a',
padding: 300
}
});
}
public highlightNextEle() {
if (this.i < this.dfs.path.length) {
this.dfs.path[this.i].addClass('highlighted');
this.i++;
setTimeout(() => {
this.highlightNextEle();
}, 1000);
}
}
onStart() {
this.i = 0;
// tslint:disable-next-line:only-arrow-functions
this.dfs = this.cy.elements().dfs('#a', function() {
}, true);
this.highlightNextEle();
}
onNodeAddFormSubmit() {
this.cy.add({
group: 'nodes',
data: {id: this.nodeAddForm.value.name},
position: {x: 300, y: 300}
},
);
}
onEdgeAddFormSubmit() {
this.cy.add({
group: 'edges',
data: {
id: this.edgeAddForm.value.source + this.edgeAddForm.value.target,
source: this.edgeAddForm.value.source,
target: this.edgeAddForm.value.target,
label: this.edgeAddForm.value.source + this.edgeAddForm.value.target
}
}
);
}
onClear() {
window.location.reload();
}
}
| 6513da4ee0ce988187fc1668fbbcb43bc6a9c3b9 | [
"TypeScript"
] | 1 | TypeScript | matsta25/depthFirstSearch | 284b630ba6690716beac727dfd7af6238d4d800d | fe7eedf5a0595e8031a8aed506bc67cf607eb09d | |
refs/heads/master | <file_sep>first_number=1
second_number=2
sum = first_number+second_number
difference = first_number-second_number
product = first_number*second_number
quotient = first_number/second_number
| d144edf7f766e10550e596d77447cded701b9467 | [
"Ruby"
] | 1 | Ruby | maddeno/programming-univbasics-3-labs-with-tdd-chi01-seng-ft-010620 | 0cfd8f349735fdc1f45fc2e7a1ec58606ee62348 | 303326becd7a5f294e65ffdc4cad15de5dce954b | |
refs/heads/master | <file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
/*By <NAME>*/
public class HealthPotion : BaseAbilityScript
{
[SerializeField]
int amount;
//Restore health to the player based on the amount the potion will give
public override void UseAbility()
{
base.UseAbility();
player.RestoreHealth(amount);
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ClimbableScript : MonoBehaviour {
[SerializeField]
Transform finalClimbingPosition;
[SerializeField]
AnimationClip myAnim;
public Transform FinalClimbingPosition
{
get { return this.finalClimbingPosition; }
}
public void OnTriggerEnter(Collider other)
{
PlayerControls player = other.gameObject.GetComponent<PlayerControls>();
if (player != null)
{
player.CurrentClimbable = this;
}
}
public void OnTriggerExit(Collider other)
{
PlayerControls player = other.gameObject.GetComponent<PlayerControls>();
if (player != null)
{
player.CurrentClimbable = null;
}
}
public void Climb(PlayerControls player)
{
player.StartCoroutine("Climb", myAnim);
//player.gameObject.transform.position = finalClimbingPosition.position;
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
/*By <NAME>*/
public class SavePointScript : MonoBehaviour, IInteractable
{
public void Reskin(Material newMat) //Byter material på savepointen för att visa att den använts
{
GetComponent<Renderer>().material = newMat;
}
public void Interact(PlayerControls player) //Sparar spelet då spelaren interagerar med scriptet
{
player.RestoreHealth(1000);
player.Stamina = 1000;
SaveManager saver = FindObjectOfType<SaveManager>();
saver.SaveGame(this.gameObject);
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
/* By <NAME>
* Följande kod är lånad från: <https://www.youtube.com/watch?v=YMj2qPq9CP8&t=350s> */
public class LoadingManager : MonoBehaviour {
public Slider progressBar;
public GameObject loadingScreen;
public Text progressText;
public void LoadScene (string scene)
{
StartCoroutine(LoadingScene(scene));
}
IEnumerator LoadingScene (string scene)
{
AsyncOperation operation = SceneManager.LoadSceneAsync(scene);
loadingScreen.SetActive(true);
while (!operation.isDone)
{
float progress = Mathf.Clamp01(operation.progress / 0.9f);
progressBar.value = progress;
progressText.text = progress * 100 + "%";
print(progressBar.value);
print(progressText.text);
print(operation.progress);
yield return null;
}
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
/*By <NAME>*/
public class CameraCollision : MonoBehaviour
{
[SerializeField]
float minDistance = 1.0f;
[SerializeField]
float maxDistance = 5.0f;
[SerializeField]
float smooth = 10.0f;
[SerializeField]
Vector3 dollyDirAdjusted;
[SerializeField]
float distance;
[SerializeField]
LayerMask layerToMask;
Vector3 dollyDir;
void Awake ()
{
dollyDir = transform.localPosition.normalized;
distance = transform.localPosition.magnitude;
}
void LateUpdate ()
{
Vector3 desiredCameraPos = transform.parent.TransformPoint(dollyDir * maxDistance);
RaycastHit hit;
//Raycast checks for objects and camera adjust when objects are hit so it does not clip through terrain
if(Physics.Linecast(transform.parent.position, desiredCameraPos, out hit, layerToMask))
{
//Clamp the distance
distance = Mathf.Clamp((hit.distance * 0.7f), minDistance, maxDistance);
}
else
{
distance = maxDistance;
}
//Lerps the cameras local position and smooths it out
transform.localPosition = Vector3.Lerp(transform.localPosition, dollyDir * distance, Time.deltaTime * smooth);
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
/*By <NAME> && <NAME>*/
public interface IKillable //Interface som används av spelaren och alla fiender samt eventuella förstörbara objekt
{
void LightAttack();
void HeavyAttack();
void TakeDamage(int damage, DamageType dmgType);
void Kill();
}
public enum MovementType //Håller koll på hur spelaren rör sig för tillfället för att tillåta och hindra spelaren att göra vissa saker
{
Idle, Walking, Sprinting, Attacking, Dodging, Dashing, Jumping, Running, Interacting, SuperJumping, Stagger
}
public class PlayerControls : MonoBehaviour, IKillable, IPausable
{
#region Serialized Variables
[Header("Player Stats")]
[Space(5)]
[SerializeField]
int maxHealth;
[SerializeField]
float maxStamina;
[SerializeField]
int leechAmount;
[SerializeField]
float maxLifeForce;
[SerializeField]
float maxPoise;
[SerializeField]
float staminaRegen;
[SerializeField]
float staminaRegenWait;
[SerializeField]
float invulnerablityTime;
[SerializeField]
float staggerTime;
[SerializeField]
float poiseCooldown;
[SerializeField]
int armor;
[SerializeField]
int leechPercentage;
[Space(10)]
[Header("Player Movement")]
[Space(5)]
[SerializeField]
float moveSpeed;
[SerializeField]
float sprintSpeed;
[SerializeField]
float jumpSpeed;
[SerializeField]
float jumpCooldown;
[SerializeField]
int rotspeed;
[Space(10)]
[Header("Player Actions")]
[Space(5)]
[SerializeField]
float dodgeCost;
[SerializeField]
float dodgeCooldown;
[SerializeField]
float dodgeLength;
[SerializeField]
float dodgeSpeed;
[SerializeField]
float attackMoveLength;
[SerializeField]
float attackCooldown;
[SerializeField]
float abilityCooldown;
[Space(10)]
[Header("Player Physics")]
[Space(5)]
[SerializeField]
float gravity;
[SerializeField]
float slopeLimit;
[SerializeField]
float slideFriction;
[SerializeField]
float safeFallDistance;
[SerializeField]
Transform playerNewGamePos;
[Space(10)]
[Header("Player Sounds")]
[Space(5)]
[SerializeField]
AudioClip swordSheathe;
[SerializeField]
AudioClip swordUnsheathe;
[SerializeField]
AudioClip lightAttack1;
[SerializeField]
AudioClip lightAttack2;
[SerializeField]
AudioClip lightAttack3;
[SerializeField]
AudioClip heavyAttack1;
[SerializeField]
AudioClip heavyAttack2;
[SerializeField]
AudioClip sandSteps;
[SerializeField]
AudioClip stoneSteps;
[SerializeField]
AudioClip woodSteps;
[SerializeField]
AudioSource rightFoot;
[SerializeField]
AudioSource leftFoot;
[SerializeField]
AudioClip landingSand;
[SerializeField]
AudioClip landingStone;
[SerializeField]
AudioClip landingWood;
[SerializeField]
float footStepsVolume;
[SerializeField]
float landingVolume;
[Space(10)]
[Header("Player Items")]
[Space(5)]
[SerializeField]
Transform weaponPosition;
[SerializeField]
SpriteRenderer currentRune;
[Space(10)]
[Header("Player Canvas")]
[Space(5)]
[SerializeField]
Slider healthBar;
[SerializeField]
Slider staminaBar;
[SerializeField]
Slider lifeForceBar;
[SerializeField]
GameObject aggroIndicator;
[SerializeField]
GameObject deathScreen;
#endregion
#region Non-Serialized Variables
CharacterController charController;
Vector3 move, dashVelocity, dodgeVelocity, hitNormal;
Vector3? dashDir, dodgeDir;
MovementType currentMovementType, previousMovementType;
PauseManager pM;
InventoryManager inventory;
IInteractable currentInteractable;
InputManager iM;
BaseWeaponScript currentWeapon;
CameraFollow cameraFollow;
GameObject weaponToEquip, lastEquippedWeapon;
ClimbableScript currentClimbable;
Animator anim;
float yVelocity, stamina, h, v, secondsUntilResetClick, attackCountdown = 0f, interactTime, dashedTime, poiseReset, poise, timeToBurn = 0f, charSpeed;
int health, lifeForce = 0, nuOfClicks = 0, abilityNo = 0;
private Transform cam;
private Vector3 camForward;
List<BaseEnemyScript> enemiesAggroing = new List<BaseEnemyScript>();
List<DamageType> resistances = new List<DamageType>();
BaseAbilityScript currentAbility;
bool inputEnabled = true, jumpMomentum = false, grounded, invulnerable = false, canDodge = true, dead = false, canSheathe = true, burning = false, frozen = false, wasGrounded,
combatStance = false, attacked = false, climbing = false, staminaRegenerating = false, staminaRegWait = false, canJump = true, fallInvulerability = false, noGravity = true; //bool shitManyBools = trueAF;
#endregion
#region Properties
//Describes which kind of movement that is currently being used
public MovementType CurrentMovementType
{
get { return this.currentMovementType; }
set { this.currentMovementType = value; }
}
public ClimbableScript CurrentClimbable
{
set { this.currentClimbable = value; }
}
public InventoryManager Inventory
{
get { return this.inventory; }
}
public BaseAbilityScript CurrentAbility
{
get { return this.currentAbility; }
}
public GameObject LastEquippedWeapon
{
get { return this.lastEquippedWeapon; }
}
public Slider StaminaBar
{
get { return this.staminaBar; }
}
public Slider LifeforceBar
{
get { return lifeForceBar; }
set { lifeForceBar = LifeforceBar; }
}
public Slider HealthBar
{
get { return healthBar; }
set { healthBar = HealthBar; }
}
//Gets and sets the current weapon
public BaseWeaponScript CurrentWeapon
{
get { return this.currentWeapon; }
}
public float Stamina
{
get { return this.stamina; }
set { this.stamina = Mathf.Clamp(value, 0f, maxStamina); staminaBar.value = stamina; }
}
public int Health
{
get { return this.health; }
set { this.health = value; if (this.health <= 0f) { Death(); } }
}
public int LifeForce
{
get { return this.lifeForce; }
set { lifeForce = value; }
}
public float YVelocity
{
set { this.yVelocity = value; }
}
public float InteractTime
{
set { interactTime = value; }
}
public Animator Anim
{
get { return anim; }
set { anim = Anim; }
}
public bool Dead
{
get { return dead; }
set { Dead = dead; }
}
#endregion
#region Main Methods
void Awake()
{
//Just setting all the variables needed
iM = FindObjectOfType<InputManager>();
charController = GetComponent<CharacterController>();
cam = FindObjectOfType<Camera>().transform;
this.health = maxHealth;
this.stamina = maxStamina;
currentMovementType = MovementType.Idle;
pM = FindObjectOfType<PauseManager>();
if (pM == null)
return;
pM.Pausables.Add(this);
inventory = gameObject.AddComponent<InventoryManager>();
slopeLimit = charController.slopeLimit;
anim = GetComponentInChildren<Animator>();
healthBar.maxValue = maxHealth;
staminaBar.maxValue = maxStamina;
lifeForceBar.maxValue = maxLifeForce;
healthBar.value = health;
staminaBar.value = stamina;
lifeForceBar.value = lifeForce;
aggroIndicator.SetActive(false);
cameraFollow = FindObjectOfType<CameraFollow>();
StartCoroutine("ZeroGravity");
}
private void Update()
{
if (inputEnabled && !dead)
{
//A sprint function which drains the stamina float upon activation
bool sprinting = false;
if (!Input.GetButton("Sprint") && currentMovementType != MovementType.Attacking && currentMovementType != MovementType.Dashing/* && currentMovementType != MovementType.Dodging*/ && currentMovementType != MovementType.Sprinting && currentMovementType != MovementType.SuperJumping && stamina < maxStamina)
{
if (staminaRegenerating)
{
stamina += (staminaRegen * Time.deltaTime); //Låter spelaren få tillbaka stamina då denne inte sprintar eller använder staminabaserade anilities
if (stamina > maxStamina)
{
stamina = maxStamina;
}
}
else if (!staminaRegWait)
{
StartCoroutine("StaminaRegenerationWait");
}
}
else
{
StopCoroutine("StaminaRegenerationWait");
staminaRegenerating = false;
staminaRegWait = false;
if (charController.isGrounded && Input.GetButton("Sprint") && stamina > 0f && move != Vector3.zero)
{
stamina -= (0.5f * Time.deltaTime);
sprinting = true;
}
}
staminaBar.value = stamina;
if (currentMovementType != MovementType.Attacking && currentMovementType != MovementType.Interacting && currentMovementType != MovementType.Stagger)
{
PlayerMovement(sprinting); //Tillåter spelaren att röra sig
}
if (!charController.isGrounded && !climbing && currentMovementType != MovementType.Interacting && !noGravity)
{
yVelocity -= gravity; //Ser till så att spelaren faller
if (yVelocity < 0)
{
anim.SetBool("Falling", true);
}
}
if (currentMovementType == MovementType.Stagger && !climbing)
{
move = Vector3.zero;
}
//Lets the character move with the character controller
if (!climbing)
charController.Move(move * Time.deltaTime);
if (currentInteractable != null && Input.GetButtonDown("Interact")) //Låter spelaren interagera med föremål i närheten som implementerar IInteractable
{
previousMovementType = currentMovementType;
currentMovementType = MovementType.Interacting;
currentInteractable.Interact(this);
this.currentInteractable = null;
move = Vector3.zero;
StartCoroutine("NonMovingInteract");
}
if (currentWeapon != null && currentWeapon.CanAttack && charController.isGrounded && this.currentWeapon != null && this.currentWeapon.CanAttack //Låter spelaren slåss
&& (currentMovementType == MovementType.Idle || currentMovementType == MovementType.Running || currentMovementType == MovementType.Sprinting || currentMovementType == MovementType.Walking || currentMovementType != MovementType.Stagger))
{
if (Input.GetAxisRaw("Fire2") < -0.5 || Input.GetButtonDown("Fire2"))
{
if (!attacked)
{
HeavyAttack();
attacked = true;
}
}
if (Input.GetButtonDown("Fire1"))
{
LightAttack();
}
}
if (attacked && (Input.GetAxisRaw("Fire2") > -0.5 || Input.GetAxisRaw("Fire2") < 0.5))
{
attacked = false;
}
if (secondsUntilResetClick > 0)
{
secondsUntilResetClick -= Time.deltaTime;
}
if (attackCountdown > 0)
{
attackCountdown -= Time.deltaTime;
}
if (poiseReset > 0)
{
poiseReset -= Time.deltaTime;
}
else
{
poise = maxPoise;
}
}
else if (!inputEnabled)
{
move = Vector3.zero;
}
}
#endregion
#region Resources
public void RestoreHealth(int amount) //Låter spelaren få tillbaka liv
{
this.health = Mathf.Clamp(this.health + amount, 0, maxHealth);
healthBar.value = health;
}
public void ReceiveLifeForce(int value) //Låter spelaren få lifeforce
{
this.lifeForce = Mathf.Clamp(this.lifeForce + value, 0, 100);
lifeForceBar.value = lifeForce;
print(lifeForce);
}
#endregion
#region Equipment
void SheatheAndUnsheathe() //Drar och stoppar undan vapen
{
if (!dead && canSheathe)
{
bool equip = weaponToEquip == null ? false : true;
anim.SetBool("WeaponDrawn", equip);
anim.SetTrigger("SheatheAndUnsheathe");
if (anim.GetBool("WeaponDrawn"))
{
SoundManager.instance.RandomizeSfx(swordSheathe, swordSheathe);
anim.SetLayerWeight(1, 1);
}
else
{
anim.SetLayerWeight(1, 0);
}
StartCoroutine("SheathingTimer");
}
}
public void Equip(GameObject equipment) //Equippar föremål
{
if (dead)
{
return;
}
if (equipment == null)
{
this.weaponToEquip = equipment;
SheatheAndUnsheathe();
return;
}
switch (equipment.GetComponent<BaseEquippableObject>().MyType)
{
case EquipableType.Ability:
if (currentAbility != null)
Destroy(currentAbility.gameObject);
currentAbility = Instantiate(equipment).GetComponent<BaseEquippableObject>() as BaseAbilityScript;
currentRune.sprite = equipment.GetComponent<BaseAbilityScript>().MyRune;
inventory.EquippedAbilityImage.sprite = equipment.GetComponent<BaseAbilityScript>().InventoryIcon;
break;
case EquipableType.Weapon:
this.weaponToEquip = equipment;
SheatheAndUnsheathe();
lastEquippedWeapon = equipment;
inventory.EquippedWeaponImage.sprite = equipment.GetComponent<BaseWeaponScript>().InventoryIcon;
break;
default:
print("unspecified object type, gör om gör rätt");
break;
}
}
public void EquipWeapon(GameObject weaponToEquip) //Code for equipping different weapons
{
if (dead)
return;
if (currentWeapon != null)
{
UnEquipWeapon();
}
this.currentWeapon = Instantiate(weaponToEquip, weaponPosition).GetComponent<BaseWeaponScript>();
this.currentWeapon.Equipper = this;
FindObjectOfType<SaveManager>().CheckIfUpgraded(this.currentWeapon);
}
public void UnEquipWeapon()
{
Destroy(this.currentWeapon.gameObject);
this.currentWeapon = null;
}
#endregion
#region Combat
//Damage to player
public void TakeDamage(int incomingDamage, DamageType dmgType) //Gör så att spelaren kan ta skada och att olika saker händer beroende på vilken typ av skada det är
{
if (dead || invulnerable)
{
return;
}
else
{
int finalDamage = ModifyDamage(incomingDamage, dmgType);
if (finalDamage <= 0)
{
return;
}
switch (dmgType)
{
case DamageType.Fire:
StopCoroutine("Burn");
StartCoroutine(Burn(5f, finalDamage / 2));
break;
case DamageType.Frost:
StopCoroutine("Freeze");
StartCoroutine(Freeze(5f));
break;
case DamageType.AutoStagger:
StartCoroutine("Stagger");
break;
case DamageType.Falling:
if (fallInvulerability)
return;
break;
}
health -= finalDamage;
healthBar.value = health;
poise -= incomingDamage;
if (incomingDamage < health && poise < incomingDamage)
{
StartCoroutine("Stagger");
}
}
if (health <= 0)
{
Death();
}
else
{
StartCoroutine("Invulnerability");
}
}
public void EnemyAggro(BaseEnemyScript enemy, bool aggroing) //Låter spelaren veta att en fiende upptäckt spelaren
{
if (aggroing)
{
enemiesAggroing.Add(enemy);
}
else
{
enemiesAggroing.Remove(enemy);
}
if (enemiesAggroing.Count > 0)
{
aggroIndicator.SetActive(true);
}
else
aggroIndicator.SetActive(false);
}
public void LightAttack() //Sets the current movement type as attacking and which attack move thats used
{
if (charController.isGrounded && grounded && attackCountdown <= 0f)
{
this.currentWeapon.Attack(1f, false);
this.currentWeapon.StartCoroutine("AttackCooldown");
attackCooldown = 0.5f;
currentWeapon.CurrentSpeed = 0.5f;
if (secondsUntilResetClick <= 0)
{
nuOfClicks = 0;
}
Mathf.Clamp(nuOfClicks, 0, 3);
nuOfClicks++;
if (nuOfClicks == 1)
{
anim.SetTrigger("LightAttack1");
secondsUntilResetClick = 1.5f;
}
if (nuOfClicks == 2)
{
anim.SetTrigger("LightAttack2");
secondsUntilResetClick = 1.5f;
}
if (nuOfClicks == 3)
{
anim.SetTrigger("LightAttack3");
nuOfClicks = 0;
attackCooldown = 1f;
currentWeapon.CurrentSpeed = 1f;
}
SoundManager.instance.RandomizeSfx(lightAttack1, lightAttack2);
move = Vector3.zero;
move += transform.forward * attackMoveLength;
attackCountdown = attackCooldown;
}
}
public void HeavyAttack() //Sets the current movement type as attacking and which attack move thats used
{
if (charController.isGrounded && grounded && attackCountdown <= 0f)
{
currentMovementType = MovementType.Attacking;
attackCooldown = 0.5f;
currentWeapon.CurrentSpeed = 0.5f;
if (secondsUntilResetClick <= 0)
{
nuOfClicks = 0;
}
Mathf.Clamp(nuOfClicks, 0, 2);
nuOfClicks++;
if (nuOfClicks == 1)
{
anim.SetTrigger("HeavyAttack1");
secondsUntilResetClick = 1.5f;
}
if (nuOfClicks == 2 || nuOfClicks == 3)
{
anim.SetTrigger("HeavyAttack2");
nuOfClicks = 0;
attackCooldown = 1f;
}
SoundManager.instance.RandomizeSfx(heavyAttack1, heavyAttack2);
move = Vector3.zero;
attackCountdown = attackCooldown;
StartCoroutine("HeavyAttackWait");
}
}
IEnumerator HeavyAttackWait()
{
yield return new WaitForSeconds(0.5f);
this.currentWeapon.Attack(1.5f, true);
this.currentWeapon.StartCoroutine("AttackCooldown");
}
public void Leech(int damageDealt) //Om spelaren slåss med ett vapen med leech får denne tillbaka 10% av skadan som liv
{
RestoreHealth(((damageDealt / 10) * leechAmount));
float floatDmg = damageDealt;
RestoreHealth(Mathf.RoundToInt(floatDmg / 100f) * leechPercentage);
}
int ModifyDamage(int damage, DamageType dmgType) //Modifies damage depending on armor, resistance etc
{
if (dmgType == DamageType.Physical)
{
damage -= armor;
}
else
foreach (DamageType resistance in resistances)
{
if (dmgType == resistance)
{
damage /= 2;
break;
}
}
return damage;
}
public void Kill() //Dödar spelaren
{
if (!dead)
{
Death();
}
}
void Death() //Kallas när spelaren dör, via skada eller Kill()
{
dead = true;
healthBar.value = 0f;
if (hitNormal.y > 0)
{
anim.SetTrigger("RightDead");
}
else if (hitNormal.y < 0)
{
anim.SetTrigger("LeftDead");
}
iM.SetInputMode(InputMode.Paused);
deathScreen.SetActive(true);
}
#endregion
#region Systems
public void PauseMe(bool pausing) //Ser till att spelaren
{
inputEnabled = !pausing;
}
#endregion
#region Movement
public void PlayerMovement(bool sprinting) //Sköter spelarens rörelser
{
if (climbing)
return;
// Gets the movement axis' for character controller and assigns them to variables
h = Input.GetAxis("Horizontal");
v = Input.GetAxis("Vertical");
//Creates a vector3 to change the character controllers forward to the direction of the camera
camForward = Vector3.Scale(cam.forward, new Vector3(1, 0, 1).normalized);
move = v * camForward + h * cam.right;
if (move.magnitude > 0.0000001f && currentMovementType != MovementType.Dashing && currentMovementType != MovementType.Dodging)
{
dashDir = null;
move *= moveSpeed;
if (sprinting || jumpMomentum)
{
move *= sprintSpeed;
}
//Changes the character models rotation to be in the direction its moving
if (!cameraFollow.LockOn)
{
anim.SetLayerWeight(2, 0);
transform.rotation = Quaternion.LookRotation(move);
}
else if (cameraFollow.LockOn)
{
transform.LookAt(cameraFollow.LookAtMe.transform);
transform.rotation = new Quaternion(0f, transform.rotation.y, 0f, transform.rotation.w);
anim.SetLayerWeight(1, 0);
anim.SetLayerWeight(2, 1);
}
}
charSpeed = CalculateSpeed(charController.velocity);
if (currentMovementType != MovementType.Dodging && currentMovementType != MovementType.Dashing && currentMovementType != MovementType.SuperJumping)
{
anim.SetFloat("Speed", charSpeed);
anim.SetFloat("SpeedX", h);
anim.SetFloat("SpeedZ", v);
if (charSpeed < 1 && currentMovementType != MovementType.Jumping)
{
currentMovementType = MovementType.Idle;
}
else if (charSpeed >= 1 && charSpeed < 5 && currentMovementType != MovementType.Jumping)
{
currentMovementType = MovementType.Walking;
}
else if (charSpeed >= 5 && charSpeed < 15 && currentMovementType != MovementType.Jumping)
{
currentMovementType = MovementType.Running;
}
}
//If the player character is on the ground you can jump
if (charController.isGrounded && canJump)
{
if (Input.GetButtonDown("Jump") && grounded && iM.CurrentInputMode == InputMode.Playing && currentMovementType != MovementType.Dashing && currentMovementType != MovementType.Dodging
&& currentMovementType != MovementType.Attacking && currentMovementType != MovementType.Interacting && currentMovementType != MovementType.Stagger)
{
if (currentClimbable != null)
{
currentClimbable.Climb(this);
}
else
{
if (sprinting)
{
jumpMomentum = true;
}
canJump = false;
yVelocity = jumpSpeed;
anim.SetTrigger("Jump");
currentMovementType = MovementType.Jumping;
}
}
}
move.y += (yVelocity * Time.deltaTime);
//If the player character is on the ground you may dodge/roll/evade as a way to avoid something
if (charController.isGrounded)
{
if (Input.GetButtonDown("Dodge") && currentMovementType != MovementType.Jumping && canJump)
{
if (stamina >= dodgeCost && canDodge)
{
stamina -= dodgeCost;
staminaBar.value = stamina;
anim.SetTrigger("Dodge");
StartCoroutine("Dodge");
StartCoroutine("DodgeCooldown");
}
}
}
//Using the character transforms' forward direction to assign which direction to dash and then moves the character in the direction with higher velocity than normal
if (currentMovementType == MovementType.Dodging)
{
if (dodgeDir == null)
{
dodgeVelocity = transform.forward * dodgeSpeed;
move += dodgeVelocity;
dodgeDir = move;
}
else
{
move = (Vector3)dodgeDir;
}
}
//Using the character transforms' forward direction to assign which direction to dash and then moves the character in the direction with high velocity
if (currentMovementType == MovementType.Dashing)
{
if (dashDir == null)
{
dashVelocity = transform.forward * 3;
move.y = 0;
move += dashVelocity * Time.deltaTime;
dashDir = move;
dashedTime = 0f;
}
else
{
move = (Vector3)dashDir;
if (dashedTime < 2f)
{
dashedTime += Time.deltaTime;
}
else
{
currentMovementType = MovementType.Idle;
}
}
}
if (!grounded && hitNormal.y >= 0f) //Får spelaren att glida ned för branta ytor
{
float xVal = -hitNormal.y * hitNormal.x * slideFriction;
float zVal = -hitNormal.y * hitNormal.z * slideFriction;
move.x += xVal;
move.z += zVal;
}
//If the angle of the object hit by the character controller collider is less or equal to the slopelimit you are grounded and wont slide down
grounded = (Vector3.Angle(Vector3.up, hitNormal) <= slopeLimit);
if (!wasGrounded && charController.isGrounded && currentMovementType != MovementType.Dodging && currentMovementType != MovementType.Dashing) //När spelaren landar efter ett hopp
{
StartCoroutine("JumpCooldown");
Landing();
anim.SetBool("Falling", false);
if (move.y < -safeFallDistance)
{
//TakeDamage(Mathf.Abs(Mathf.RoundToInt((move.y * 3f) + safeFallDistance)), DamageType.Falling); //FallDamage, avstängd för att den dödar spelaren utan att spelaren faktiskt faller då vissa av spelarens handlingar/animationer får spelaren att inte räknas som grounded
}
jumpMomentum = false;
currentMovementType = MovementType.Idle;
}
if (sprinting && charController.velocity.magnitude > 0f && currentMovementType != MovementType.Jumping && currentMovementType != MovementType.Dodging && currentMovementType != MovementType.Dashing)
{
currentMovementType = MovementType.Sprinting;
}
wasGrounded = charController.isGrounded;
anim.SetBool("Land", charController.isGrounded);
}
float CalculateSpeed(Vector3 velocity) //Räknar ut vilken fart spelaren har så att animatorn kan spela upp rätt animation
{
Vector3 newVelocity = new Vector3(velocity.x, 0f, velocity.z);
return newVelocity.magnitude;
}
#endregion
#region Sound Events
void Footsteps() //Spelar upp fotstegsljud då spelaren går
{
if (charController.isGrounded && grounded && move != Vector3.zero)
{
RaycastHit hit;
if (Physics.Raycast(transform.position, Vector3.down, out hit))
{
if (hit.collider.gameObject.tag == "Sand")
{
leftFoot.volume = footStepsVolume;
leftFoot.PlayOneShot(sandSteps);
}
else if (hit.collider.gameObject.tag == "Stone")
{
leftFoot.volume = footStepsVolume;
leftFoot.PlayOneShot(stoneSteps);
}
else if (hit.collider.gameObject.tag == "Wood")
{
leftFoot.volume = footStepsVolume;
leftFoot.PlayOneShot(woodSteps);
}
}
}
}
void Landing() //Spelar upp ett ljud då spelaren landar efter ett hopp
{
RaycastHit hit;
if (Physics.Raycast(transform.position, Vector3.down, out hit))
{
if (hit.collider.gameObject.tag == "Sand")
{
leftFoot.volume = landingVolume;
rightFoot.volume = landingVolume;
leftFoot.PlayOneShot(landingSand);
rightFoot.PlayOneShot(landingSand);
}
else if (hit.collider.gameObject.tag == "Stone")
{
leftFoot.volume = landingVolume;
rightFoot.volume = landingVolume;
leftFoot.PlayOneShot(landingStone);
rightFoot.PlayOneShot(landingStone);
}
else if (hit.collider.gameObject.tag == "Wood")
{
leftFoot.volume = landingVolume;
rightFoot.volume = landingVolume;
leftFoot.PlayOneShot(landingWood);
rightFoot.PlayOneShot(landingWood);
}
}
}
#endregion
#region Colliders
void OnTriggerEnter(Collider other) //Avgör vilken IIinteractable spelaren kan interagera med
{
if (other.gameObject.GetComponent<IInteractable>() != null)
currentInteractable = other.gameObject.GetComponent<IInteractable>();
}
void OnTriggerExit(Collider other)
{
IInteractable otherInteractable = other.gameObject.GetComponent<IInteractable>();
if (otherInteractable != null && currentInteractable == otherInteractable)
currentInteractable = null;
}
void OnControllerColliderHit(ControllerColliderHit hit) //Hjälper till att få spelaren att glida ned för branta ytor
{
hitNormal = hit.normal;
}
#endregion
#region Coroutines
public IEnumerator ZeroGravity()
{
yield return new WaitForSeconds(3);
noGravity = false;
}
public IEnumerator Climb(AnimationClip climbAnim) //Får spelaren att kunna klättra upp för vissa ytor
{
ClimbableScript currentClimb = currentClimbable;
gameObject.transform.LookAt(currentClimbable.FinalClimbingPosition);
gameObject.transform.rotation = new Quaternion(0f, transform.rotation.y, 0f, transform.rotation.w);
this.climbing = true;
string animTrigger = (climbAnim.length > 2f) ? "Climb1" : "Climb2";
anim.SetTrigger(animTrigger);
yield return new WaitForSeconds(climbAnim.length - 0.15f);
gameObject.transform.position = currentClimb.FinalClimbingPosition.position;
this.climbing = false;
}
public IEnumerator AbilityCooldown() //Hindrar spelaren från att använda abilities under en tid efter att en ability använts
{
BaseAbilityScript.CoolingDown = true;
yield return new WaitForSeconds(abilityCooldown);
BaseAbilityScript.CoolingDown = false;
}
IEnumerator DodgeCooldown() //Hindrar spelaren från att hoppa eller använda sin dodge under en tid efter att spelaren hoppat eller dodgen använts
{
if (!dead)
{
canDodge = false;
canJump = false;
yield return new WaitForSeconds(dodgeCooldown);
canJump = true;
canDodge = true;
}
}
IEnumerator Dodge() //Enumerator smooths out the dodge/roll/evade so it doesn't happen instantaneously
{
if (!dead)
{
currentMovementType = MovementType.Dodging;
yield return new WaitForSeconds(dodgeLength);
currentMovementType = MovementType.Running;
dodgeDir = null;
}
}
IEnumerator NonMovingInteract() //Hindrar spelaren från att röra sig medan denne interagerar med något
{
canJump = false;
yield return new WaitForSeconds(interactTime);
currentMovementType = MovementType.Idle;
canJump = true;
}
IEnumerator SheathingTimer() //Spawnar och despawnar vapen efter en viss tid för att matcha med animationer
{
if (!dead)
{
canSheathe = false;
yield return new WaitForSeconds(0.4f);
if (weaponToEquip != null)
{
SoundManager.instance.RandomizeSfx(swordUnsheathe, swordUnsheathe);
EquipWeapon(weaponToEquip);
}
else if (currentWeapon != null)
{
UnEquipWeapon();
}
canSheathe = true;
}
}
IEnumerator Invulnerability() //Hindrar spelaren från att ta skada under en viss tid
{
invulnerable = true;
yield return new WaitForSeconds(invulnerablityTime);
invulnerable = false;
}
public IEnumerator PreventFallDamage() //Hindrar spelaren från att ta fallskada under en viss tid
{
fallInvulerability = true;
yield return new WaitForSeconds(5f);
fallInvulerability = false;
}
IEnumerator Stagger()
{
currentMovementType = MovementType.Stagger;
anim.SetTrigger("Stagger");
poiseReset = poiseCooldown;
yield return new WaitForSeconds(staggerTime);
currentMovementType = MovementType.Idle;
}
protected IEnumerator Burn(float burnDuration, int burnDamage) //Gör så att spelaren tar eldskada under en viss tid
{
burning = true;
timeToBurn += burnDuration;
while (timeToBurn > 0f)
{
yield return new WaitForSeconds(0.5f);
this.health -= burnDamage;
timeToBurn -= Time.deltaTime;
}
timeToBurn = 0f;
burning = false;
}
protected IEnumerator Freeze(float freezeTime) //Får spelaren att röra sig långsammare under en viss tid
{
if (!frozen)
{
frozen = true;
float originalSpeed = moveSpeed;
yield return new WaitForSeconds(freezeTime);
moveSpeed = originalSpeed;
frozen = false;
}
}
IEnumerator StaminaRegenerationWait() //Väntar en stund innan spelaren kan återfå stamina
{
staminaRegWait = true;
yield return new WaitForSeconds(staminaRegenWait);
staminaRegWait = false;
staminaRegenerating = true;
}
IEnumerator JumpCooldown() //Hindrar spelaren från att hoppa under en viss tid efter att denne hoppat
{
yield return new WaitForSeconds(jumpCooldown);
canJump = true;
}
#endregion
}<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
/*By <NAME> && <NAME>*/
public class MagicForceJump : BaseAbilityScript
{
[SerializeField]
GameObject effectsPrefab, spawnPos;
[SerializeField]
float magicJumpSpeed, delayTime;
public override void UseAbility()
{
if (player.GetComponent<CharacterController>().isGrounded)
{
base.UseAbility();
//instantiate a magic jump circle
StartCoroutine("SuperJump");
player.CurrentMovementType = MovementType.SuperJumping;
}
}
protected override void Update()
{
if ((player.CurrentMovementType == MovementType.Idle
|| player.CurrentMovementType == MovementType.Sprinting //Låter spelaren använda abilities när den inte attackerar, dodgar eller liknande
|| player.CurrentMovementType == MovementType.Walking
|| player.CurrentMovementType == MovementType.Jumping)
&& Input.GetButtonDown("Ability")
&& !coolingDown && !player.Dead)
{
if (player.Stamina >= abilityCost) //Får magicforcejump att dra stamina istället för lifeforce
{
player.StaminaBar.value = player.Stamina;
UseAbility();
}
}
}
IEnumerator SuperJump()
{
GameObject jumpParticles = Instantiate(effectsPrefab, spawnPos.transform.position, spawnPos.transform.rotation);
player.Anim.SetTrigger("SuperJump");
yield return new WaitForSeconds(delayTime);
//Add a force to the player going up form your current position.
player.YVelocity = magicJumpSpeed;
Destroy(jumpParticles, 1.5f);
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
/*By <NAME>*/
public class TerrainDeformTracks : MonoBehaviour
{
[SerializeField]
Shader drawShader;
[SerializeField]
GameObject rightFoot, leftFoot;
[SerializeField, Range(0, 500)]
float brushSize;
[SerializeField, Range(-5, 5)]
float brushStrength;
private RenderTexture splatMap;
private Material sandMaterial, drawMaterial, fadeMat;
private RaycastHit hit;
RenderTexture temp;
Vector4 footstepCoordinate;
//List<FootstepsRemoval> removeSteps = new List<FootstepsRemoval>();
//public List<FootstepsRemoval> RemoveSteps
//{
// get { return this.removeSteps; }
//}
// Use this for initialization
void Start()
{
drawMaterial = new Material(drawShader);
//fadeMat = new Material(reverseShader);
drawMaterial.SetVector("_Color", Color.red);
//fadeMat.SetVector("_Color", Color.black);
}
//void Update()
//{
// foreach(FootstepsRemoval step in removeSteps)
// {
// step.Fade();
// }
//}
void RightFootDeform()
{
if (Physics.Raycast(rightFoot.gameObject.transform.position, Vector3.down, out hit))
{
if (hit.transform.CompareTag("Sand"))
{
if (sandMaterial == null)
{
sandMaterial = hit.transform.GetComponent<Terrain>().materialTemplate;
sandMaterial.SetTexture("_Splat", splatMap = new RenderTexture(1024, 1024, 0, RenderTextureFormat.ARGBFloat));
}
drawMaterial.SetVector("_Coordinate", footstepCoordinate = new Vector4(hit.textureCoord.x, hit.textureCoord.y, 0, 0));
drawMaterial.SetFloat("_Strength", brushStrength);
drawMaterial.SetFloat("_Size", brushSize);
temp = RenderTexture.GetTemporary(splatMap.width, splatMap.height, 0, RenderTextureFormat.ARGBFloat);
Graphics.Blit(splatMap, temp);
Graphics.Blit(temp, splatMap, drawMaterial);
//drawMaterial.SetVector("_Color", Color.black);
//
//FootstepsRemoval fader = new FootstepsRemoval(this, splatMap, temp, footstepCoordinate, fadeMat, brushStrength);
//drawMaterial.SetVector("_Color", Color.red);
////fadeMat.SetFloat("_Strength", drawMaterial.GetFloat("_Strength") * -0.001f);
////fadeMat.SetVector("_Color", Color.black);
//FootstepsRemoval remover = Instantiate((Resources.Load("FootprintRemover") as GameObject).GetComponent<FootstepsRemoval>());
//remover.RemovePrint(this, splatMap, temp, footstepCoordinate, fadeMat, brushStrength);
RenderTexture.ReleaseTemporary(temp);
//Instantiate(footstepRemover, hit.transform.position, hit.transform.rotation);
}
}
}
void LeftFootDeform()
{
if (Physics.Raycast(leftFoot.gameObject.transform.position, Vector3.down, out hit))
{
if (hit.transform.CompareTag("Sand"))
{
if (sandMaterial == null)
{
sandMaterial = hit.transform.GetComponent<Terrain>().materialTemplate;
sandMaterial.SetTexture("_Splat", splatMap = new RenderTexture(1024, 1024, 0, RenderTextureFormat.ARGBFloat));
}
drawMaterial.SetVector("_Coordinate", footstepCoordinate = new Vector4(hit.textureCoord.x, hit.textureCoord.y, 0, 0));
drawMaterial.SetFloat("_Strength", brushStrength);
drawMaterial.SetFloat("_Size", brushSize);
temp = RenderTexture.GetTemporary(splatMap.width, splatMap.height, 0, RenderTextureFormat.ARGBFloat);
Graphics.Blit(splatMap, temp);
Graphics.Blit(temp, splatMap, drawMaterial);
//drawMaterial.SetVector("_Color", Color.black);
//Material fadeMat = new Material(drawShader);
//FootstepsRemoval fader = new FootstepsRemoval(this, splatMap, temp, footstepCoordinate, fadeMat, brushStrength);
//drawMaterial.SetVector("_Color", Color.red);
////fadeMat.SetFloat("_Strength", drawMaterial.GetFloat("_Strength") * -0.001f);
////fadeMat.SetVector("_Color", Color.black);
//FootstepsRemoval remover = Instantiate((Resources.Load("FootprintRemover") as GameObject).GetComponent<FootstepsRemoval>());
//remover.RemovePrint(this, splatMap, temp, footstepCoordinate, fadeMat, brushStrength);
RenderTexture.ReleaseTemporary(temp);
//Instantiate(footstepRemover, hit.transform.position, hit.transform.rotation);
}
}
}
//IEnumerator RemoveFootstep()
//{
// yield return new WaitForSeconds(3);
// drawMaterial.SetVector("_Coordinate", footstepCoordinate);
// drawMaterial.SetVector("_Color", Color.black);
// drawMaterial.SetFloat("_Strength", brushStrength);
// drawMaterial.SetFloat("_Size", brushSize);
// temp = RenderTexture.GetTemporary(splatMap.width, splatMap.height, 0, RenderTextureFormat.ARGBFloat);
// Graphics.Blit(splatMap, temp);
// Graphics.Blit(temp, splatMap, drawMaterial);
// drawMaterial.SetVector("_Color", Color.red);
//}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
/*By <NAME> && <NAME>*/
public enum DamageType //Olika typer av skada som kan hanteras på olika sätt och göra olika saker
{
Physical, Frost, Fire, Falling, Leech, AutoStagger
}
public enum AttackMoves
{
QuickAttack, StrongAttack, Sideswipe, PiercingAttack
}
public enum AttackMoveSets
{
LightWeapon, HeavyWeapon
}
public enum Upgrade //Olika uppgraderingstyper
{
None, DamageUpgrade, FireUpgrade, FrostUpgrade, LeechUpgrade
}
public class BaseWeaponScript : BaseEquippableObject
{
[SerializeField]
protected int origninalLightDamage, originalHeavyDamage, lightDamage;
protected int heavyDamage;
[SerializeField]
protected float attackSpeed;
[SerializeField]
protected AttackMoves[] attacks;
[SerializeField]
protected DamageType dmgType;
[SerializeField]
protected AudioClip enemyHit1, enemyHit2, enemyHit3, swing1, swing2, thrust;
protected bool canAttack = true, heavy = false;
protected MovementType previousMovement;
protected Upgrade currentUpgrade = Upgrade.None;
protected float currentSpeed;
protected int upgradeLevel = 0;
protected Collider myColl;
public int UpgradeLevel
{
get { return this.upgradeLevel; }
}
public Upgrade CurrentUpgrade
{
get { return this.currentUpgrade; }
}
public float CurrentSpeed
{
get { return this.currentSpeed; }
set { this.currentSpeed = value; StartCoroutine("ResetSpeed"); }
}
public bool CanAttack
{
get { return this.canAttack; }
}
public float AttackSpeed
{
get { return this.attackSpeed; }
}
public AttackMoves[] Attacks
{
get { return this.attacks; }
}
protected IKillable equipper;
public IKillable Equipper
{
set { if (this.equipper == null) this.equipper = value; }
get { return this.equipper; }
}
protected override void Start()
{
base.Start();
this.myColl = GetComponent<Collider>();
this.currentSpeed = attackSpeed;
if (this.lightDamage == 0)
{
this.lightDamage = origninalLightDamage;
this.heavyDamage = originalHeavyDamage;
}
this.equipper = GetComponentInParent<IKillable>();
this.myColl.enabled = false;
}
public void Attack(float attackTime, bool heavy) //Håller koll på om vapnet ska göra light eller heavy skada
{
if (!canAttack)
return;
this.heavy = heavy;
StartCoroutine(AttackMove(attackTime));
}
protected IEnumerator AttackMove(float attackTime) //Tillåter vapnet att göra skada under tiden det svingas
{
myColl.enabled = true;
yield return new WaitForSeconds(attackTime);
myColl.enabled = false;
}
public IEnumerator AttackCooldown() //Hindrar vapnet från att attackera under en viss tid efter det attackerat
{
this.canAttack = false;
if (equipper is PlayerControls)
{
previousMovement = player.CurrentMovementType;
player.CurrentMovementType = MovementType.Attacking;
yield return new WaitForSeconds(currentSpeed);
player.CurrentMovementType = previousMovement;
}
else if (equipper is BaseEnemyScript)
{
previousMovement = (equipper as BaseEnemyScript).CurrentMovementType;
(equipper as BaseEnemyScript).CurrentMovementType = MovementType.Attacking;
yield return new WaitForSeconds(currentSpeed);
(equipper as BaseEnemyScript).CurrentMovementType = previousMovement;
}
else
{
print("nu gick nåt åt helvete");
}
this.canAttack = true;
if (equipper is PlayerControls)
(equipper as PlayerControls).CurrentMovementType = MovementType.Idle;
else
(equipper as BaseEnemyScript).CurrentMovementType = MovementType.Idle;
}
public void ApplyUpgrade(Upgrade upgrade) //Uppgraderar vapnet
{
if (this.currentUpgrade != Upgrade.DamageUpgrade)
this.upgradeLevel = 0;
this.currentUpgrade = upgrade;
if (upgrade == Upgrade.DamageUpgrade && upgradeLevel < 3)
{
upgradeLevel++;
this.lightDamage += lightDamage / 2;
this.heavyDamage += heavyDamage / 2;
}
else
{
this.upgradeLevel = 1;
switch (upgrade)
{
case Upgrade.FireUpgrade:
this.dmgType = DamageType.Fire;
break;
case Upgrade.FrostUpgrade:
this.dmgType = DamageType.Frost;
break;
case Upgrade.LeechUpgrade:
this.dmgType = DamageType.Leech;
break;
default:
print("Nu blev nåt fel här");
break;
}
this.lightDamage = origninalLightDamage;
this.heavyDamage = originalHeavyDamage;
}
}
//Deals damage to an object with IKillable on it.
public virtual void DealDamage(IKillable target)
{
int damage = (heavy == true ? heavyDamage : lightDamage);
target.TakeDamage(damage, dmgType);
}
protected IEnumerator ResetSpeed()
{
yield return new WaitForSeconds(1f);
this.currentSpeed = attackSpeed;
}
//When a weapon hits a killable target the script triggers and deals damage to target
public void OnTriggerEnter(Collider other)
{
if ((equipper is BaseEnemyScript && (equipper as BaseEnemyScript).CurrentMovementType == MovementType.Attacking)
|| (equipper is PlayerControls && (equipper as PlayerControls).CurrentMovementType == MovementType.Attacking))
{
IKillable targetToHit = other.gameObject.GetComponent<IKillable>();
if (targetToHit == null || (equipper is BaseEnemyScript && targetToHit is BaseEnemyScript) || (equipper is PlayerControls && targetToHit is PlayerControls))
{
return;
}
DealDamage(targetToHit);
SoundManager.instance.RandomizeSfx(enemyHit1, enemyHit2, enemyHit3);
}
}
}<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
/*By <NAME>*/
public class BaseEquippableObject : MonoBehaviour { //Script som alla föremål som kan ligga i inventoryt ärver från
[SerializeField]
protected Sprite inventoryIcon;
[SerializeField]
protected string objectName;
[SerializeField]
protected EquipableType myType;
protected PlayerControls player;
protected bool equipped = false;
public string ObjectName
{
get { return this.objectName; }
}
public EquipableType MyType
{
get { return this.myType; }
}
public Sprite InventoryIcon
{
get { return this.inventoryIcon; }
}
protected virtual void Start()
{
this.player = FindObjectOfType<PlayerControls>();
}
}<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
/*By <NAME> && <NAME>*/
public class MagicDash : BaseAbilityScript
{
[SerializeField]
float duration;
public override void UseAbility() //Activated from the BaseAbility script. If the player have enough stamina the ability will activate and drain the staminaCost
{
base.UseAbility();
player.Anim.SetTrigger("Dash");
StartCoroutine("Dash");
}
protected override void Update()
{
if ((player.CurrentMovementType == MovementType.Idle
|| player.CurrentMovementType == MovementType.Sprinting //Låter spelaren använda abilities när den inte attackerar, dodgar eller liknande
|| player.CurrentMovementType == MovementType.Walking
|| player.CurrentMovementType == MovementType.Jumping)
&& Input.GetButtonDown("Ability")
&& !coolingDown && !player.Dead)
{
if (player.Stamina >= abilityCost) //Gör så att MagicDash drar stamina istället för lifeforce
{
player.StaminaBar.value = player.Stamina;
UseAbility();
}
}
}
IEnumerator Dash() //Enumerator smooths out the dash so it doesn't happen instantaneously
{
player.CurrentMovementType = MovementType.Dashing;
yield return new WaitForSeconds(duration);
player.CurrentMovementType = MovementType.Running;
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
/*By <NAME> && <NAME>*/
public class OpenDoor : MonoBehaviour, IInteractable
{
[SerializeField]
GameObject doorToOpen, leverPullPos;
[SerializeField]
float movePlayerSmoother;
[SerializeField]
AudioClip openGate;
float leverPullPosX;
Animator anim, animDoor;
PlayerControls playerToMove;
MovementType previousMovement;
void Start()
{
anim = this.GetComponent<Animator>();
animDoor = doorToOpen.gameObject.GetComponent<Animator>();
}
public void Interact(PlayerControls player) //Spelar upp en animation medan spelaren drar i en spak för att öppna en dörr
{
playerToMove = player;
StartCoroutine("MovePlayerToInteract");
player.InteractTime = 5.13f;
anim.SetTrigger("LeverPull");
playerToMove.Anim.SetTrigger("PullLever");
StartCoroutine("OpenSesame");
}
IEnumerator OpenSesame() //Öppnar den relevanta dörren
{
yield return new WaitForSeconds(5.0f);
SoundManager.instance.PlaySingle(openGate);
animDoor.SetTrigger("OpenDoor");
}
IEnumerator MovePlayerToInteract() //Flyttar spelaren till rätt position medan animationen spelas
{
float t = 0;
while (t < 1)
{
t += Time.deltaTime / movePlayerSmoother;
//playerToMove.Anim.SetFloat("Speed", 0.5f);
playerToMove.gameObject.transform.position = Vector3.Lerp(playerToMove.gameObject.transform.position, leverPullPos.gameObject.transform.position, t);
playerToMove.gameObject.transform.rotation = Quaternion.Lerp(playerToMove.gameObject.transform.rotation, leverPullPos.gameObject.transform.rotation, t);
yield return null;
}
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
/*By <NAME>*/
public class DynamicSceneManager : MonoBehaviour
{
[SerializeField]
private string masterSceneTriggers;
[SerializeField]
private string terrainScene;
[SerializeField]
private string startArea;
[SerializeField]
private string bridgeScene;
public static DynamicSceneManager instance { get; set; }
private void Awake() //Laddar in de områden som ska laddas då spelet startar
{
Application.backgroundLoadingPriority = ThreadPriority.Low;
instance = this;
StartCoroutine(Load(masterSceneTriggers));
StartCoroutine(Load(terrainScene));
StartCoroutine(Load(startArea));
StartCoroutine(Load(bridgeScene));
}
public IEnumerator Load(string sceneName) //Laddar en scen additivt så att den är aktiv tillsammans med redan aktiva scener
{
if (!SceneManager.GetSceneByName(sceneName).isLoaded)
{
yield return SceneManager.LoadSceneAsync(sceneName, LoadSceneMode.Additive);
}
}
public IEnumerator UnLoad(string sceneName) //Stänger av en aktiv scen
{
if (SceneManager.GetSceneByName(sceneName).isLoaded)
{
yield return SceneManager.UnloadSceneAsync(sceneName);
}
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
/*By <NAME>*/
public class BaseAbilityScript : BaseEquippableObject //Ett script alla magic abilities ärver från
{
[SerializeField]
protected int abilityCost;
[SerializeField]
protected Sprite myRune;
protected static bool coolingDown = false;
public static bool CoolingDown
{
get { return coolingDown; }
set { coolingDown = value; }
}
public Sprite MyRune
{
get { return this.myRune; }
}
public virtual void UseAbility() //Virtuell metod som overrideas av alla abilities så att de faktiskt gör olika saker
{
player.StartCoroutine("AbilityCooldown"); //Startar en cooldown när spelaren använder en ability
}
protected virtual void Update()
{
if ((player.CurrentMovementType == MovementType.Idle
|| player.CurrentMovementType == MovementType.Sprinting //Låter spelaren använda abilities när den inte attackerar, dodgar eller liknande
|| player.CurrentMovementType == MovementType.Walking
|| player.CurrentMovementType == MovementType.Jumping)
&& Input.GetButtonDown("Ability")
&& !coolingDown && !player.Dead)
{
if (player.LifeForce >= abilityCost) //Drar resurser för att använda abilities
{
player.LifeForce -= abilityCost;
player.LifeforceBar.value = player.LifeForce;
}
else
{
player.Health -= abilityCost;
player.HealthBar.value = player.Health;
}
UseAbility();
}
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
/*By <NAME>*/
public class MagicProjectile : BaseAbilityScript
{
public GameObject magicProjectilePrefab;
public Camera cameraPosition;
//A currently public float,
//so you can adjust the speed as necessary.
public float speed = 20.0f;
public override void UseAbility()
{
base.UseAbility();
//instantiate a magic projectile
GameObject magicProjectile = Instantiate(magicProjectilePrefab, transform.position, transform.rotation);
//Add a force to the magic going forward form your current position.
magicProjectile.GetComponent<Rigidbody>().AddForce(magicProjectile.transform.forward * speed, ForceMode.Impulse);
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
/*By <NAME>*/
public interface IInteractable
{
void Interact(PlayerControls player);
}
public class PickUpable : MonoBehaviour, IInteractable
{
[SerializeField]
GameObject item;
public void Interact(PlayerControls player) //Låter spelaren plocka upp ett föremål och lägga det i inventoryt
{
player.InteractTime = 2f;
player.Anim.SetTrigger("PickUp");
StartCoroutine("DetachItem");
player.Inventory.NewEquippable(item);
Destroy(this.gameObject, 2);
}
IEnumerator DetachItem() //Tar bort föremålet från sin parent för att kunna ta bort föremålet från världen utan att hindra spelaren från att läggadet i sitt inventory
{
yield return new WaitForSeconds(1.9f);
item.transform.parent = null;
item.transform.position = Vector3.zero;
item.transform.rotation = new Quaternion(0f, 0f, 0f, item.transform.rotation.w);
}
}
| 19d0490474b0ff1f002f2b969cea53895234d512 | [
"C#"
] | 16 | C# | regrets123/Winner-of-SGA | 3da5cb60ec63a7c91949d97a02e0a4580c26f898 | 260d255009d963c01d6927cd3a5eed65fba129de | |
refs/heads/main | <repo_name>LeQuangVinh0201/VaccineProject<file_sep>/QLVaccine/src/main/java/com/softech/FrameApp/ui/User/Home.java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.softech.FrameApp.ui.User;
/**
*
* @author leduc
*/
public class Home extends javax.swing.JPanel {
/**
* Creates new form Home
*/
public Home() {
initComponents();
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
jpnHome = new javax.swing.JPanel();
jpnPersonalInfo = new javax.swing.JPanel();
jLabel7 = new javax.swing.JLabel();
jLabel8 = new javax.swing.JLabel();
jSeparator4 = new javax.swing.JSeparator();
jpnCertification = new javax.swing.JPanel();
jLabel5 = new javax.swing.JLabel();
jLabel6 = new javax.swing.JLabel();
jSeparator3 = new javax.swing.JSeparator();
jpnSchedule = new javax.swing.JPanel();
jLabel3 = new javax.swing.JLabel();
jLabel4 = new javax.swing.JLabel();
jSeparator2 = new javax.swing.JSeparator();
jpnHome.setBackground(new java.awt.Color(204, 255, 204));
jpnPersonalInfo.setBackground(new java.awt.Color(0, 204, 204));
jpnPersonalInfo.addMouseListener(new java.awt.event.MouseAdapter() {
public void mouseClicked(java.awt.event.MouseEvent evt) {
jpnPersonalInfoMouseClicked(evt);
}
});
jLabel7.setIcon(new javax.swing.ImageIcon(getClass().getResource("/com/softech/appqlsv/icons/calendar (1).png"))); // NOI18N
jLabel8.setFont(new java.awt.Font("Arial", 1, 24)); // NOI18N
jLabel8.setForeground(new java.awt.Color(255, 255, 255));
jLabel8.setText("Thông tin cá nhân");
jSeparator4.setOrientation(javax.swing.SwingConstants.VERTICAL);
javax.swing.GroupLayout jpnPersonalInfoLayout = new javax.swing.GroupLayout(jpnPersonalInfo);
jpnPersonalInfo.setLayout(jpnPersonalInfoLayout);
jpnPersonalInfoLayout.setHorizontalGroup(
jpnPersonalInfoLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jpnPersonalInfoLayout.createSequentialGroup()
.addGap(27, 27, 27)
.addComponent(jLabel7)
.addGap(18, 18, 18)
.addComponent(jSeparator4, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel8)
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
jpnPersonalInfoLayout.setVerticalGroup(
jpnPersonalInfoLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jpnPersonalInfoLayout.createSequentialGroup()
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addGroup(jpnPersonalInfoLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, jpnPersonalInfoLayout.createSequentialGroup()
.addGroup(jpnPersonalInfoLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(jSeparator4, javax.swing.GroupLayout.PREFERRED_SIZE, 128, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel7))
.addGap(32, 32, 32))
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, jpnPersonalInfoLayout.createSequentialGroup()
.addComponent(jLabel8)
.addGap(83, 83, 83))))
);
jpnCertification.setBackground(new java.awt.Color(153, 153, 255));
jLabel5.setIcon(new javax.swing.ImageIcon(getClass().getResource("/com/softech/appqlsv/icons/calendar (1).png"))); // NOI18N
jLabel6.setFont(new java.awt.Font("Arial", 1, 24)); // NOI18N
jLabel6.setForeground(new java.awt.Color(255, 255, 255));
jLabel6.setText("Chứng nhận ");
jSeparator3.setOrientation(javax.swing.SwingConstants.VERTICAL);
javax.swing.GroupLayout jpnCertificationLayout = new javax.swing.GroupLayout(jpnCertification);
jpnCertification.setLayout(jpnCertificationLayout);
jpnCertificationLayout.setHorizontalGroup(
jpnCertificationLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jpnCertificationLayout.createSequentialGroup()
.addGap(27, 27, 27)
.addComponent(jLabel5)
.addGap(18, 18, 18)
.addComponent(jSeparator3, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(jLabel6)
.addContainerGap(54, Short.MAX_VALUE))
);
jpnCertificationLayout.setVerticalGroup(
jpnCertificationLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jpnCertificationLayout.createSequentialGroup()
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addGroup(jpnCertificationLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, jpnCertificationLayout.createSequentialGroup()
.addGroup(jpnCertificationLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(jSeparator3, javax.swing.GroupLayout.PREFERRED_SIZE, 128, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel5))
.addGap(32, 32, 32))
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, jpnCertificationLayout.createSequentialGroup()
.addComponent(jLabel6)
.addGap(83, 83, 83))))
);
jpnSchedule.setBackground(new java.awt.Color(255, 102, 153));
jLabel3.setIcon(new javax.swing.ImageIcon(getClass().getResource("/com/softech/appqlsv/icons/calendar (1).png"))); // NOI18N
jLabel4.setFont(new java.awt.Font("Arial", 1, 24)); // NOI18N
jLabel4.setForeground(new java.awt.Color(255, 255, 255));
jLabel4.setText("<NAME>");
jSeparator2.setOrientation(javax.swing.SwingConstants.VERTICAL);
javax.swing.GroupLayout jpnScheduleLayout = new javax.swing.GroupLayout(jpnSchedule);
jpnSchedule.setLayout(jpnScheduleLayout);
jpnScheduleLayout.setHorizontalGroup(
jpnScheduleLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jpnScheduleLayout.createSequentialGroup()
.addGap(27, 27, 27)
.addComponent(jLabel3)
.addGap(18, 18, 18)
.addComponent(jSeparator2, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel4)
.addContainerGap(105, Short.MAX_VALUE))
);
jpnScheduleLayout.setVerticalGroup(
jpnScheduleLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jpnScheduleLayout.createSequentialGroup()
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addGroup(jpnScheduleLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(jSeparator2, javax.swing.GroupLayout.PREFERRED_SIZE, 128, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel3))
.addGap(32, 32, 32))
.addGroup(jpnScheduleLayout.createSequentialGroup()
.addGap(59, 59, 59)
.addComponent(jLabel4)
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
javax.swing.GroupLayout jpnHomeLayout = new javax.swing.GroupLayout(jpnHome);
jpnHome.setLayout(jpnHomeLayout);
jpnHomeLayout.setHorizontalGroup(
jpnHomeLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jpnHomeLayout.createSequentialGroup()
.addGap(56, 56, 56)
.addComponent(jpnPersonalInfo, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(46, 46, 46)
.addComponent(jpnSchedule, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(51, 51, 51)
.addComponent(jpnCertification, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap(62, Short.MAX_VALUE))
);
jpnHomeLayout.setVerticalGroup(
jpnHomeLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jpnHomeLayout.createSequentialGroup()
.addGap(20, 20, 20)
.addGroup(jpnHomeLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jpnCertification, javax.swing.GroupLayout.PREFERRED_SIZE, 152, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jpnPersonalInfo, javax.swing.GroupLayout.PREFERRED_SIZE, 152, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jpnSchedule, javax.swing.GroupLayout.PREFERRED_SIZE, 152, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(746, Short.MAX_VALUE))
);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jpnHome, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jpnHome, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
);
}// </editor-fold>//GEN-END:initComponents
private void jpnPersonalInfoMouseClicked(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_jpnPersonalInfoMouseClicked
}//GEN-LAST:event_jpnPersonalInfoMouseClicked
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel4;
private javax.swing.JLabel jLabel5;
private javax.swing.JLabel jLabel6;
private javax.swing.JLabel jLabel7;
private javax.swing.JLabel jLabel8;
private javax.swing.JSeparator jSeparator2;
private javax.swing.JSeparator jSeparator3;
private javax.swing.JSeparator jSeparator4;
private javax.swing.JPanel jpnCertification;
private javax.swing.JPanel jpnHome;
private javax.swing.JPanel jpnPersonalInfo;
private javax.swing.JPanel jpnSchedule;
// End of variables declaration//GEN-END:variables
}
<file_sep>/QLVaccine/src/main/java/com/softech/FrameApp/ui/Login_Register/RegisterUser.java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.softech.FrameApp.ui.Login_Register;
import com.softech.Login.userDAO;
import com.softech.Login.userLogin;
import java.awt.Color;
import javax.swing.JOptionPane;
/**
*
* @author leduc
*/
public class RegisterUser extends javax.swing.JPanel {
/**
* Creates new form RegisterUser1
*/
public RegisterUser() {
initComponents();
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
jpnRegister = new javax.swing.JPanel();
jLabel3 = new javax.swing.JLabel();
jLabel4 = new javax.swing.JLabel();
txtEmail = new javax.swing.JTextField();
jLabel5 = new javax.swing.JLabel();
jLabel8 = new javax.swing.JLabel();
jLabel9 = new javax.swing.JLabel();
jLabel6 = new javax.swing.JLabel();
jLabel7 = new javax.swing.JLabel();
txtPassword = new javax.swing.JPasswordField();
txtfullName = new javax.swing.JTextField();
txtConfirmPassword = new <PASSWORD>.swing.JPasswordField();
jSeparator1 = new javax.swing.JSeparator();
btnRegister = new javax.swing.JButton();
jSeparator2 = new javax.swing.JSeparator();
txtPhoneNumber = new javax.swing.JFormattedTextField();
setPreferredSize(new java.awt.Dimension(650, 470));
jLabel3.setFont(new java.awt.Font("Arial", 1, 30)); // NOI18N
jLabel3.setHorizontalAlignment(javax.swing.SwingConstants.CENTER);
jLabel3.setText("Đăng ký");
jLabel4.setFont(new java.awt.Font("Arial", 1, 18)); // NOI18N
jLabel4.setHorizontalAlignment(javax.swing.SwingConstants.CENTER);
jLabel4.setText("Vui lòng cung cấp thông tin chính xác");
txtEmail.setFont(new java.awt.Font("Times New Roman", 0, 14)); // NOI18N
txtEmail.addKeyListener(new java.awt.event.KeyAdapter() {
public void keyPressed(java.awt.event.KeyEvent evt) {
txtEmailKeyPressed(evt);
}
});
jLabel5.setFont(new java.awt.Font("Arial", 1, 18)); // NOI18N
jLabel5.setText("Họ và tên:");
jLabel8.setFont(new java.awt.Font("Arial", 1, 18)); // NOI18N
jLabel8.setText("Số điện thoại:");
jLabel9.setFont(new java.awt.Font("Arial", 1, 18)); // NOI18N
jLabel9.setText("Email:");
jLabel6.setFont(new java.awt.Font("Arial", 1, 18)); // NOI18N
jLabel6.setText("Mật khẩu:");
jLabel7.setFont(new java.awt.Font("Arial", 1, 18)); // NOI18N
jLabel7.setText("Xác nhận mật khẩu");
txtPassword.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
txtPasswordActionPerformed(evt);
}
});
txtPassword.addKeyListener(new java.awt.event.KeyAdapter() {
public void keyPressed(java.awt.event.KeyEvent evt) {
txtPasswordKeyPressed(evt);
}
});
txtfullName.setFont(new java.awt.Font("Times New Roman", 0, 14)); // NOI18N
txtfullName.addKeyListener(new java.awt.event.KeyAdapter() {
public void keyPressed(java.awt.event.KeyEvent evt) {
txtfullNameKeyPressed(evt);
}
});
txtConfirmPassword.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
txtConfirmPasswordActionPerformed(evt);
}
});
txtConfirmPassword.addKeyListener(new java.awt.event.KeyAdapter() {
public void keyPressed(java.awt.event.KeyEvent evt) {
txtConfirmPasswordKeyPressed(evt);
}
});
btnRegister.setFont(new java.awt.Font("Arial", 1, 24)); // NOI18N
btnRegister.setText("Đăng ký");
btnRegister.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnRegisterActionPerformed(evt);
}
});
try {
txtPhoneNumber.setFormatterFactory(new javax.swing.text.DefaultFormatterFactory(new javax.swing.text.MaskFormatter("##########")));
} catch (java.text.ParseException ex) {
ex.printStackTrace();
}
javax.swing.GroupLayout jpnRegisterLayout = new javax.swing.GroupLayout(jpnRegister);
jpnRegister.setLayout(jpnRegisterLayout);
jpnRegisterLayout.setHorizontalGroup(
jpnRegisterLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, jpnRegisterLayout.createSequentialGroup()
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(btnRegister, javax.swing.GroupLayout.PREFERRED_SIZE, 144, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(139, 139, 139))
.addGroup(jpnRegisterLayout.createSequentialGroup()
.addContainerGap()
.addGroup(jpnRegisterLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel3, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jLabel4, javax.swing.GroupLayout.DEFAULT_SIZE, 417, Short.MAX_VALUE)
.addGroup(jpnRegisterLayout.createSequentialGroup()
.addGroup(jpnRegisterLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jSeparator1)
.addComponent(txtConfirmPassword)
.addComponent(txtPassword)
.addComponent(txtEmail, javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(txtfullName)
.addComponent(jSeparator2, javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(jpnRegisterLayout.createSequentialGroup()
.addGroup(jpnRegisterLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel5)
.addComponent(jLabel8)
.addComponent(jLabel9)
.addComponent(jLabel6)
.addComponent(jLabel7))
.addGap(0, 0, Short.MAX_VALUE))
.addComponent(txtPhoneNumber))
.addContainerGap())))
);
jpnRegisterLayout.setVerticalGroup(
jpnRegisterLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jpnRegisterLayout.createSequentialGroup()
.addGap(16, 16, 16)
.addComponent(jLabel3)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(jLabel4, javax.swing.GroupLayout.PREFERRED_SIZE, 25, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jSeparator1, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(21, 21, 21)
.addComponent(jLabel5)
.addGap(18, 18, 18)
.addComponent(txtfullName, javax.swing.GroupLayout.PREFERRED_SIZE, 32, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(jLabel8)
.addGap(18, 18, 18)
.addComponent(txtPhoneNumber, javax.swing.GroupLayout.PREFERRED_SIZE, 32, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(jLabel9)
.addGap(18, 18, 18)
.addComponent(txtEmail, javax.swing.GroupLayout.PREFERRED_SIZE, 32, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(jLabel6)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(txtPassword, javax.swing.GroupLayout.PREFERRED_SIZE, 37, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(25, 25, 25)
.addComponent(jLabel7)
.addGap(18, 18, 18)
.addComponent(txtConfirmPassword, javax.swing.GroupLayout.PREFERRED_SIZE, 37, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(16, 16, 16)
.addComponent(jSeparator2, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(btnRegister)
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jpnRegister, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jpnRegister, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
);
}// </editor-fold>//GEN-END:initComponents
private void txtfullNameKeyPressed(java.awt.event.KeyEvent evt) {//GEN-FIRST:event_txtfullNameKeyPressed
txtfullName.setBackground(Color.white);
}//GEN-LAST:event_txtfullNameKeyPressed
private void btnRegisterActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnRegisterActionPerformed
try {
userLogin admin = new userLogin();
admin.setUsername(txtPhoneNumber.getText());
admin.setPassword(new String(txtPassword.getPassword()));
admin.setFullname(txtfullName.getText());
admin.setEmail(txtEmail.getText());
String username = txtPhoneNumber.getText();
String fullname = txtfullName.getText();
String comfirmP = new String(txtConfirmPassword.getPassword());
String password = new String(txtPassword.getPassword());
String email = txtEmail.getText();
if (username.equals("")) {
JOptionPane.showMessageDialog(this, "vui lòng điền thông tin");
txtPhoneNumber.setBackground(Color.red);
txtfullName.setBackground(Color.red);
txtEmail.setBackground(Color.red);
txtPassword.setBackground(Color.red);
txtConfirmPassword.setBackground(Color.red);
} else {
if (comfirmP.equals(password)) {
if (email.contains("<EMAIL>")) {
userDAO dao = new userDAO();
dao.insert(admin);
JOptionPane.showMessageDialog(this, "đăng ký thành công!");
} else {
txtEmail.setBackground(Color.red);
JOptionPane.showMessageDialog(this, "email không đúng");
}
} else {
txtConfirmPassword.setBackground(Color.red);
JOptionPane.showMessageDialog(this, "password is correct");
}
}
} catch (Exception e) {
e.printStackTrace();
JOptionPane.showMessageDialog(this, "Error :" + e);
}
}//GEN-LAST:event_btnRegisterActionPerformed
private void txtEmailKeyPressed(java.awt.event.KeyEvent evt) {//GEN-FIRST:event_txtEmailKeyPressed
txtEmail.setBackground(Color.white);
}//GEN-LAST:event_txtEmailKeyPressed
private void txtPasswordKeyPressed(java.awt.event.KeyEvent evt) {//GEN-FIRST:event_txtPasswordKeyPressed
txtPassword.setBackground(Color.white);
}//GEN-LAST:event_txtPasswordKeyPressed
private void txtPasswordActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_txtPasswordActionPerformed
// TODO add your handling code here:
}//GEN-LAST:event_txtPasswordActionPerformed
private void txtConfirmPasswordKeyPressed(java.awt.event.KeyEvent evt) {//GEN-FIRST:event_txtConfirmPasswordKeyPressed
txtConfirmPassword.setBackground(Color.white);
}//GEN-LAST:event_txtConfirmPasswordKeyPressed
private void txtConfirmPasswordActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_txtConfirmPasswordActionPerformed
// TODO add your handling code here:
}//GEN-LAST:event_txtConfirmPasswordActionPerformed
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton btnRegister;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel4;
private javax.swing.JLabel jLabel5;
private javax.swing.JLabel jLabel6;
private javax.swing.JLabel jLabel7;
private javax.swing.JLabel jLabel8;
private javax.swing.JLabel jLabel9;
private javax.swing.JSeparator jSeparator1;
private javax.swing.JSeparator jSeparator2;
private javax.swing.JPanel jpnRegister;
private javax.swing.JPasswordField txtConfirmPassword;
private javax.swing.JTextField txtEmail;
private javax.swing.JPasswordField txtPassword;
private javax.swing.JFormattedTextField txtPhoneNumber;
private javax.swing.JTextField txtfullName;
// End of variables declaration//GEN-END:variables
}
<file_sep>/QLVaccine/src/main/java/com/softech/user/dao/NguoiDanDao.java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.softech.user.dao;
import com.softech.ConnectDB.connectDbManagerVaccine;
import com.softech.FrameApp.ui.Login_Register.Login;
import com.softech.user.model.NguoiDan;
import java.sql.Blob;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import javax.sql.rowset.serial.SerialBlob;
/**
*
* @author PC
*/
public class NguoiDanDao {
public boolean update(NguoiDan nd)
throws Exception {
String sql = "UPDATE dbo.NguoiTiem"+ " SET name = ?,gender = ?,dateOfBirth = ?,identification_ID = ?, bhyt_number = ?, email= ?, address = ?, image = ?"
+ " WHERE userName_phoneNumber = ?";
try (
Connection con = connectDbManagerVaccine.OpenConnection(); PreparedStatement pstmt = con.prepareStatement(sql);) {
pstmt.setString(1, nd.getName());
pstmt.setInt(2, nd.getGender());
pstmt.setString(3, nd.getDateOfBirth());
pstmt.setString(4, nd.getIdentification_ID());
pstmt.setString(5, nd.getBhyt_number());
pstmt.setString(6, nd.getEmail());
pstmt.setString(7, nd.getAddress());
pstmt.setString(9, nd.getUserName_phoneNumber());
if (nd.getImage()!= null) {
Blob hinh = new SerialBlob(nd.getImage());
pstmt.setBlob(8, hinh);
} else {
Blob hinh = null;
pstmt.setBlob(8, hinh);
}
return pstmt.executeUpdate() > 0; // neu lon hon 0 return true, nguoc lai return false
}
}
public boolean delete(String userName_phoneNumber)
throws Exception {
// phai co 1 khoang trang sau " , khong thoi se loi sql
String sql = "delete from VaccineApp" +
" where userName_phoneNumber = ?";
try (
Connection con = connectDbManagerVaccine.OpenConnection();
PreparedStatement pstmt = con.prepareStatement(sql);
){
pstmt.setString(1, userName_phoneNumber);
return pstmt.executeUpdate() > 0; // neu lon hon 0 return true, nguoc lai return false
}
}
public NguoiDan findByUserName(String userName)
throws Exception {
// phai co 1 khoang trang sau " , khong thoi se loi sql
String sql = "select *, CONVERT(varchar,NguoiTiem.dateOfBirth,103) as newDateOfBirth"
+ " from NguoiTiem where UserName_phoneNumber = ?";
try (
Connection con = connectDbManagerVaccine.OpenConnection();
PreparedStatement pstmt = con.prepareStatement(sql);
){
pstmt.setString(1, userName);
try(ResultSet rs = pstmt.executeQuery()){
if(rs.next()){
NguoiDan nd = new NguoiDan();
nd.setName(rs.getString("name"));
nd.setDateOfBirth(rs.getString("newDateOfBirth"));
nd.setIdentification_ID(rs.getString("identification_ID"));
nd.setEmail(rs.getString("email"));
nd.setAddress(rs.getString("address"));
nd.setGender(rs.getInt("gender"));
nd.setBhyt_number(rs.getString("bhyt_number"));
Blob blob =rs.getBlob("image");
if(blob != null){
nd.setImage(blob.getBytes(1, (int) blob.length()));
}
return nd;
}
}
return null;
}
}
public int getCertificattion(String userName)
throws Exception {
// phai co 1 khoang trang sau " , khong thoi se loi sql
String sql = "select * from NguoiTiem, LichTiem where UserName_phoneNumber = ?";
try (
Connection con = connectDbManagerVaccine.OpenConnection();
PreparedStatement pstmt = con.prepareStatement(sql);
){
pstmt.setString(1, userName);
try(ResultSet rs = pstmt.executeQuery()){
int max = 0;
while (rs.next()) {
if (rs.getInt("confirmation") == 0) {
if (max > 0) {
return max;
} else {
max = 0;
}
} else {
if (rs.getInt("shot") == 1) {
max = 1;
} else {
max = 2;
break;
}
}
}
return max;
}
}
}
}
<file_sep>/QLVaccine/src/main/java/com/softech/FrameApp/ui/Login_Register/Login.java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.softech.FrameApp.ui.Login_Register;
import com.softech.FrameApp.ui.Admin.MainJFrameAdmin;
import com.softech.FrameApp.ui.User.MainJFrameUser;
import com.softech.Login.userDAO;
import com.softech.Login.userLogin;
import java.awt.Color;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JTabbedPane;
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*//**
*
* @author leduc
*/
public class Login extends javax.swing.JPanel {
private userDAO udao = new userDAO();
public static String username_verified;
/**
* Creates new form RegisterUser1
*/
public Login() {
initComponents();
}
public Login(JFrame jFrame, boolean b) {
throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates.
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
jPanel1 = new javax.swing.JPanel();
jLabel3 = new javax.swing.JLabel();
jLabel4 = new javax.swing.JLabel();
jSeparator1 = new javax.swing.JSeparator();
jLabel6 = new javax.swing.JLabel();
jLabel8 = new javax.swing.JLabel();
btnRegister = new javax.swing.JButton();
jSeparator2 = new javax.swing.JSeparator();
txtPassword = new javax.swing.JPasswordField();
jSeparator3 = new javax.swing.JSeparator();
jcheckAdmin = new javax.swing.JCheckBox();
txtUserName = new javax.swing.JFormattedTextField();
setPreferredSize(new java.awt.Dimension(427, 743));
jPanel1.setBackground(new java.awt.Color(102, 255, 204));
jLabel3.setFont(new java.awt.Font("Arial", 1, 30)); // NOI18N
jLabel3.setHorizontalAlignment(javax.swing.SwingConstants.CENTER);
jLabel3.setText("Đăng nhập");
jLabel4.setFont(new java.awt.Font("Arial", 1, 18)); // NOI18N
jLabel4.setHorizontalAlignment(javax.swing.SwingConstants.CENTER);
jLabel4.setText("Vui lòng cung cấp thông tin chính xác");
jSeparator1.setBackground(new java.awt.Color(0, 0, 0));
jLabel6.setFont(new java.awt.Font("Arial", 1, 18)); // NOI18N
jLabel6.setText("Mật khẩu:");
jLabel8.setFont(new java.awt.Font("Arial", 1, 18)); // NOI18N
jLabel8.setText("Số điện thoại:");
btnRegister.setFont(new java.awt.Font("Arial", 1, 24)); // NOI18N
btnRegister.setText("Đăng nhập");
btnRegister.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnRegisterActionPerformed(evt);
}
});
txtPassword.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
txtPasswordActionPerformed(evt);
}
});
txtPassword.addKeyListener(new java.awt.event.KeyAdapter() {
public void keyPressed(java.awt.event.KeyEvent evt) {
txtPasswordKeyPressed(evt);
}
});
jSeparator3.setBackground(new java.awt.Color(0, 0, 0));
jcheckAdmin.setFont(new java.awt.Font("Arial", 0, 14)); // NOI18N
jcheckAdmin.setText("Truy cập quyền Admin");
jcheckAdmin.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jcheckAdminActionPerformed(evt);
}
});
try {
txtUserName.setFormatterFactory(new javax.swing.text.DefaultFormatterFactory(new javax.swing.text.MaskFormatter("##########")));
} catch (java.text.ParseException ex) {
ex.printStackTrace();
}
txtUserName.addMouseListener(new java.awt.event.MouseAdapter() {
public void mouseExited(java.awt.event.MouseEvent evt) {
txtUserNameMouseExited(evt);
}
});
txtUserName.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
txtUserNameActionPerformed(evt);
}
});
txtUserName.addKeyListener(new java.awt.event.KeyAdapter() {
public void keyPressed(java.awt.event.KeyEvent evt) {
txtUserNameKeyPressed(evt);
}
});
javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1);
jPanel1.setLayout(jPanel1Layout);
jPanel1Layout.setHorizontalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(jSeparator2, javax.swing.GroupLayout.PREFERRED_SIZE, 7, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(0, 0, Short.MAX_VALUE))
.addGroup(jPanel1Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(btnRegister, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jSeparator1)
.addComponent(jLabel4, javax.swing.GroupLayout.DEFAULT_SIZE, 407, Short.MAX_VALUE)
.addComponent(txtUserName)
.addComponent(txtPassword)
.addComponent(jLabel3, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jSeparator3)
.addGroup(jPanel1Layout.createSequentialGroup()
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel8)
.addComponent(jLabel6))
.addGap(0, 0, Short.MAX_VALUE))))
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, jPanel1Layout.createSequentialGroup()
.addGap(0, 0, Short.MAX_VALUE)
.addComponent(jcheckAdmin)))
.addContainerGap())
);
jPanel1Layout.setVerticalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addGap(45, 45, 45)
.addComponent(jLabel3)
.addGap(29, 29, 29)
.addComponent(jLabel4, javax.swing.GroupLayout.PREFERRED_SIZE, 25, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(jSeparator1, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(jLabel8)
.addGap(18, 18, 18)
.addComponent(txtUserName, javax.swing.GroupLayout.PREFERRED_SIZE, 41, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(jLabel6)
.addGap(18, 18, 18)
.addComponent(txtPassword, javax.swing.GroupLayout.PREFERRED_SIZE, 37, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(40, 40, 40)
.addComponent(jcheckAdmin)
.addGap(18, 18, 18)
.addComponent(jSeparator3, javax.swing.GroupLayout.PREFERRED_SIZE, 25, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 112, Short.MAX_VALUE)
.addComponent(btnRegister)
.addGap(37, 37, 37)
.addComponent(jSeparator2, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(76, 76, 76))
);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(jPanel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(jPanel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addContainerGap())
);
}// </editor-fold>//GEN-END:initComponents
private void btnRegisterActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnRegisterActionPerformed
try {
if (txtUserName.getText().equals("")) {
txtUserName.setBackground(Color.red);
txtPassword.setBackground(Color.red);
} else {
if (jcheckAdmin.isSelected()) {
userLogin admin = udao.findbyAdmin(txtUserName.getText());
if (admin != null) {
String password = new String(txtPassword.getPassword());
String passwordCurrent = admin.getPassword();
if (passwordCurrent.equals(password)) {
JOptionPane.showMessageDialog(this, "đăng nhập thành công");
MainJFrameAdmin mainJFrameAdmin = new MainJFrameAdmin();
mainJFrameAdmin.setVisible(true);
mainJFrameAdmin.pack();
mainJFrameAdmin.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
} else {
JOptionPane.showMessageDialog(this, "tài khoản hoặc đăng nhập không đúng !");
}
} else {
JOptionPane.showMessageDialog(this, "tài khoản hoặc đăng nhập không đúng !");
}
} else {
userLogin user = udao.findbyUser(txtUserName.getText());
if (user != null) {
String password = new String(txtPassword.getPassword());
String passwordCurrent = user.getPassword();
if (passwordCurrent.equals(password)) {
JOptionPane.showMessageDialog(this, "đăng nhập thành công");
MainJFrameUser mainJFrameUser = new MainJFrameUser();
mainJFrameUser.setVisible(true);
mainJFrameUser.pack();
mainJFrameUser.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
username_verified = user.getUsername();
} else {
JOptionPane.showMessageDialog(this, "tài khoản hoặc đăng nhập không đúng !");
}
} else {
JOptionPane.showMessageDialog(this, "tài khoản hoặc đăng nhập không đúng !");
}
}
}
} catch (Exception e) {
e.printStackTrace();
JOptionPane.showMessageDialog(this, "Error :" + e);
}
}//GEN-LAST:event_btnRegisterActionPerformed
private void btnCancelActionPerformed(java.awt.event.ActionEvent evt) {
JOptionPane.showConfirmDialog(this, "bạn có muốn thoát không ? ");
System.exit(0);
}
private void txtPasswordKeyPressed(java.awt.event.KeyEvent evt) {//GEN-FIRST:event_txtPasswordKeyPressed
txtPassword.setBackground(Color.white);
}//GEN-LAST:event_txtPasswordKeyPressed
private void txtPasswordActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_txtPasswordActionPerformed
// TODO add your handling code here:
}//GEN-LAST:event_txtPasswordActionPerformed
private void jcheckAdminActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jcheckAdminActionPerformed
// TODO add your handling code here:
}//GEN-LAST:event_jcheckAdminActionPerformed
private void txtUserNameKeyPressed(java.awt.event.KeyEvent evt) {//GEN-FIRST:event_txtUserNameKeyPressed
txtPassword.setBackground(Color.white);
}//GEN-LAST:event_txtUserNameKeyPressed
private void txtUserNameActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_txtUserNameActionPerformed
// TODO add your handling code here:
}//GEN-LAST:event_txtUserNameActionPerformed
private void txtUserNameMouseExited(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_txtUserNameMouseExited
// TODO add your handling code here:
}//GEN-LAST:event_txtUserNameMouseExited
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton btnRegister;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel4;
private javax.swing.JLabel jLabel6;
private javax.swing.JLabel jLabel8;
private javax.swing.JPanel jPanel1;
private javax.swing.JSeparator jSeparator1;
private javax.swing.JSeparator jSeparator2;
private javax.swing.JSeparator jSeparator3;
private javax.swing.JCheckBox jcheckAdmin;
private javax.swing.JPasswordField txtPassword;
private javax.swing.JFormattedTextField txtUserName;
// End of variables declaration//GEN-END:variables
}
<file_sep>/README.md
# VaccineProject
create vaccine app
<file_sep>/QLVaccine/pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.softech</groupId>
<artifactId>QLVaccine</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>jar</packaging>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.source>15</maven.compiler.source>
<maven.compiler.target>15</maven.compiler.target>
</properties>
<dependencies>
<!-- https://mvnrepository.com/artifact/com.microsoft.sqlserver/mssql-jdbc -->
<dependency>
<groupId>com.microsoft.sqlserver</groupId>
<artifactId>mssql-jdbc</artifactId>
<version>9.4.0.jre11</version>
</dependency>
<dependency>
<groupId>commons-codec</groupId>
<artifactId>commons-codec</artifactId>
<version>1.15</version>
</dependency>
<dependency>
<groupId>com.sun.mail</groupId>
<artifactId>javax.mail</artifactId>
<version>1.6.2</version>
</dependency>
</dependencies>
<name>QLVaccine</name>
</project><file_sep>/QLVaccine/src/main/java/com/softech/FrameApp/ui/User/LichTiem.java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.softech.FrameApp.ui.User;
import com.softech.ConnectDB.connectDbManagerVaccine;
import com.softech.FrameApp.ui.Login_Register.Login;
import com.softech.user.dao.NguoiDanDao;
import com.softech.user.model.NguoiDan;
import java.sql.Blob;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.List;
import javax.swing.table.DefaultTableModel;
/**
*
* @author leduc
*/
public class LichTiem extends javax.swing.JPanel {
private DefaultTableModel tblModel;
/**
* Creates new form LichTiem1
*/
public LichTiem() {
initComponents();
initTable();
loadSchedule();
}
private void initTable(){
tblModel = new DefaultTableModel();
tblModel.setColumnIdentifiers(new String[]{
"Họ tên","Ngày sinh","Số ĐT","Số CMND","Mũi thứ","Loại vaccine","Nhà sản xuất","Lịch tiêm"
});
tblSchedule.setModel(tblModel);
}
private void loadSchedule(){
try {
String sql = "select NguoiTiem.name, CONVERT(varchar,NguoiTiem.dateOfBirth,103) as dateOfBirth, NguoiTiem.userName_phoneNumber," +
" NguoiTiem.identification_ID, LichTiem.shot, Vaccine.nameOfVaccine, Vaccine.manufacturer, CONVERT(varchar,LichTiem.schedule,103) as schedule from NguoiTiem, LichTiem, Vaccine where NguoiTiem.userName_phoneNumber = LichTiem.phoneNumber_ID" +
" and LichTiem.vaccine_ID = Vaccine.vaccine_ID and NguoiTiem.userName_phoneNumber = ?";
try (
Connection con = connectDbManagerVaccine.OpenConnection();
PreparedStatement pstmt = con.prepareStatement(sql);
){
Login lg = new Login();
String userName = lg.username_verified;
pstmt.setString(1, userName);
try(ResultSet rs = pstmt.executeQuery()){
tblModel.setRowCount(0);
boolean result = rs.next();
if(!result){
NguoiDanDao dao = new NguoiDanDao();
NguoiDan nd = dao.findByUserName(userName);
tblModel.addRow(new Object[]{
nd.getName(), nd.getDateOfBirth(),userName,
nd.getIdentification_ID(), " ", " "," ", " "});
}
while(result){
tblModel.addRow(new Object[]{
rs.getString("name"), rs.getString("dateOfBirth"),rs.getString("userName_phoneNumber"),
rs.getString("identification_ID"), rs.getInt("shot"), rs.getString("nameOfVaccine"),
rs.getString("manufacturer"), rs.getString("schedule")
});
result = rs.next();
}
}
}
tblModel.fireTableDataChanged();
} catch (Exception e) {
e.printStackTrace();
}
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
jScrollPane1 = new javax.swing.JScrollPane();
tblSchedule = new javax.swing.JTable();
tblSchedule.setModel(new javax.swing.table.DefaultTableModel(
new Object [][] {
{null, null, null, null, null},
{null, null, null, null, null},
{null, null, null, null, null},
{null, null, null, null, null}
},
new String [] {
"STT", "Họ và tên", "Số điện thoại", "Ngày tiêm mũi 1", "Ngày tiêm mũi 2"
}
));
jScrollPane1.setViewportView(tblSchedule);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jScrollPane1, javax.swing.GroupLayout.DEFAULT_SIZE, 1269, Short.MAX_VALUE)
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jScrollPane1, javax.swing.GroupLayout.DEFAULT_SIZE, 646, Short.MAX_VALUE)
);
}// </editor-fold>//GEN-END:initComponents
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JTable tblSchedule;
// End of variables declaration//GEN-END:variables
}
<file_sep>/QLVaccine/src/main/java/com/softech/user/model/NguoiDan.java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.softech.user.model;
/**
*
* @author PC
*/
public class NguoiDan {
private String userName_phoneNumber, passWord, name, identification_ID, bhyt_number, email, address;
private String dateOfBirth, vaccine1_schedule, vaccine2_schedule;
private int gender;
private byte[] image;
public NguoiDan() {
}
public NguoiDan(String userName_phoneNumber, String passWord, String name, String identification_ID, String bhyt_number, String email, String address, String dateOfBirth, String vaccine1_schedule, String vaccine2_schedule, int gender, byte[] image) {
this.userName_phoneNumber = userName_phoneNumber;
this.passWord = <PASSWORD>;
this.name = name;
this.identification_ID = identification_ID;
this.bhyt_number = bhyt_number;
this.email = email;
this.address = address;
this.dateOfBirth = dateOfBirth;
this.vaccine1_schedule = vaccine1_schedule;
this.vaccine2_schedule = vaccine2_schedule;
this.gender = gender;
this.image = image;
}
public String getUserName_phoneNumber() {
return userName_phoneNumber;
}
public void setUserName_phoneNumber(String userName_phoneNumber) {
this.userName_phoneNumber = userName_phoneNumber;
}
public String getPassWord() {
return passWord;
}
public void setPassWord(String passWord) {
this.passWord = passWord;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getIdentification_ID() {
return identification_ID;
}
public void setIdentification_ID(String identification_ID) {
this.identification_ID = identification_ID;
}
public String getBhyt_number() {
return bhyt_number;
}
public void setBhyt_number(String bhyt_number) {
this.bhyt_number = bhyt_number;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getDateOfBirth() {
return dateOfBirth;
}
public void setDateOfBirth(String dateOfBirth) {
this.dateOfBirth = dateOfBirth;
}
public String getVaccine1_schedule() {
return vaccine1_schedule;
}
public void setVaccine1_schedule(String vaccine1_schedule) {
this.vaccine1_schedule = vaccine1_schedule;
}
public String getVaccine2_schedule() {
return vaccine2_schedule;
}
public void setVaccine2_schedule(String vaccine2_schedule) {
this.vaccine2_schedule = vaccine2_schedule;
}
public int getGender() {
return gender;
}
public void setGender(int gender) {
this.gender = gender;
}
public byte[] getImage() {
return image;
}
public void setImage(byte[] image) {
this.image = image;
}
}
<file_sep>/QLVaccine/src/main/java/com/softech/Login/mailPassword.java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.softech.Login;
import java.util.Properties;
import javax.mail.Address;
import javax.mail.Authenticator;
import javax.mail.Message;
import javax.mail.PasswordAuthentication;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
/**
*
* @author admin
*/
public class mailPassword {
public static void send(Email email) throws Exception {
Properties prop = new Properties();//cau hinh mail
prop.put("mail.smtp.host", "smtp.gmail.com"); //SMTP host
prop.put("mail.smtp.port", "587"); //TLS Port
prop.put("mail.smtp.auth", "true"); //enable authentication
prop.put("mail.smtp.starttls.enable", "true"); //enable
Session session = Session.getInstance(prop, new Authenticator() { // lop cho phep thuc hien cac xac thuc khi ket noi voi mail
protected PasswordAuthentication getPasswordAuthentication() { //email gui mail
return new PasswordAuthentication(email.getFrom(), email.getFromPassword());
}
});
try {
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress(email.getFrom())); // dia chi mail gui
message.setRecipients(Message.RecipientType.TO, InternetAddress.parse(email.getTo())); // danh sach ng nhan
message.setSubject(email.getSubject()); // tieu de mail
message.setContent(email.getContent(), "text/html; charset=utf-8"); // noi dung mail . kieu noi dung va kieu dinh dang
Transport.send(message);// method send mail
} catch (Exception e) {
e.printStackTrace();
throw e;
}
}
}
| 87661b383ac3f0eb1386d863bdbb5abf903a676b | [
"Markdown",
"Java",
"Maven POM"
] | 9 | Java | LeQuangVinh0201/VaccineProject | f08c0a8bc834a73a91c2939dd7f1e263105d8b86 | 275bd6f49aa148504b6a30678f60dc9067523625 | |
refs/heads/master | <repo_name>Smokbomb/videolist<file_sep>/README.md
## Installation
Step 1 docker build -t videolist .
Step 2 docker run -d -p 3000:3000 videolist
<file_sep>/src/socket/events/events.gateway.ts
import {
SubscribeMessage,
WebSocketGateway,
WebSocketServer,
WsResponse,
} from '@nestjs/websockets';
import { from, Observable } from 'rxjs';
import { map } from 'rxjs/operators';
// import { VideoService } from 'video/video.service';
@WebSocketGateway()
export class EventsGateway {
// constructor(private readonly videoService: VideoService) { }
// @WebSocketServer() server;
// @SubscribeMessage('video')
// async getVideo(client, id: string): WsResponse<any> {
// await this.videoService.updateLike(id);
// return await { 'video': await this.videoService.findAll() };
// }
}<file_sep>/src/video/video.controller.ts
import { Controller, Get, Post, Body, Param } from '@nestjs/common';
import { VideoService } from './video.service';
@Controller('video')
export class VideoController {
constructor(private readonly videoService: VideoService) { }
@Get()
async findAll(): Promise<any[]> {
return await this.videoService.findAll();
}
@Get(':id')
findName(@Param() params) {
return this.videoService.findById(params.id);
}
@Post()
savePhoto(@Body() body) {
if (body.like === '1') {
this.videoService.updateLike(body.id);
}
if (body.view === '1') {
this.videoService.updateView(body.id);
}
}
}
| 813b9cbf34e91228c82410d15c80d7c9c038877f | [
"Markdown",
"TypeScript"
] | 3 | Markdown | Smokbomb/videolist | 1b2a2a2946fe8274705a8a9109a64f29ef41f691 | 8aa31fff417431befc53f4eb303e6185d2ad611d | |
refs/heads/main | <repo_name>Kyle-Xu001/ROS-Practice<file_sep>/assignment1/src/turtle_circle.cpp
#include <ros/ros.h>
#include <turtlesim/Pose.h>
#include <geometry_msgs/Twist.h>
//callback function
void chatterCallback(const turtlesim::Pose& msg){
ROS_INFO("Turtle is at [%f, %f, %f]", (float)(msg.x), (float)(msg.y), (float)(msg.theta));
}
int main(int argc, char **argv){
ros::init(argc, argv, "turtle_circle");
ros::NodeHandle n;
ros::Publisher turtle_pub = n.advertise<geometry_msgs::Twist>("/turtle1/cmd_vel",1);
ros::Subscriber turtle_sub = n.subscribe("/turtle1/pose", 1, chatterCallback);
ros::Rate loop_rate(10);
while(ros::ok()){
geometry_msgs::Twist msg;
msg.linear.x = 1;
msg.linear.y = 0;
msg.linear.z = 0;
msg.angular.x = 0;
msg.angular.y = 0;
msg.angular.z = 1;
turtle_pub.publish(msg);
ros::spinOnce();
loop_rate.sleep();
}
return 0;
}<file_sep>/README.md
# ROS-Practice
This repository is created for programming assignments in ***ROS Theory and Practice***, which includes functional nodes and extended functions.
## Assignment1
This part is about communication programming, which includes applications on publisher, subscriber, server and client.
The function of each node is defined as follows:
**turtle_call**: Create a new turtle at specific location predefined in the code.
**turtle_circle**: Control the existed turtle in circular motion, and subscribe the pose of turtle in real time.
**turtle_control**: Control the real-time motion of existed turtle by inputting turtle name and velocity parameters in command line. (*Achieved by **Publisher** function*)
**turtle_spawn**: Create a new turtle at a random location with the name defined in command line. (*Achieved by single **Client** function*)
**turtle_spawn_client / turtle_spawn_server**: Create a new turtle at a random location with the name defined in command line. (*Achieved by combination of **Server** and **Client***)
<file_sep>/assignment1/src/turtle_call.cpp
#include <ros/ros.h>
#include <turtlesim/Spawn.h>
int main(int argc, char **argv){
ros::init(argc, argv, "turtle_call");
ros:: NodeHandle n;
ros::service::waitForService("/spawn");
ros::ServiceClient client = n.serviceClient<turtlesim::Spawn>("/spawn");
turtlesim::Spawn srv;
srv.request.x = 5.0;
srv.request.y = 5.0;
srv.request.theta = 0;
srv.request.name = "Bob";
ROS_INFO("Call service to spawn a turtle at [%f, %f], name %s", (float)(srv.request.x), (float)(srv.request.y), srv.request.name.c_str());
client.call(srv);
ROS_INFO("Turtle Spawn: OK!");
return 0;
}<file_sep>/assignment1/src/turtle_spawn.cpp
#include <ros/ros.h>
#include <turtlesim/Spawn.h>
#include <iostream>
#include <ctime>
int main(int argc, char **argv){
//Set the real random seed
srand((unsigned int)time(NULL));
//Initiate the ROS node
ros::init(argc, argv, "turtle_spawn");
ros::NodeHandle n;
//Construct the service client to call service "spawn"
ros::service::waitForService("/spawn");
ros::ServiceClient client = n.serviceClient<turtlesim::Spawn>("/spawn");
//Define the request msg
turtlesim::Spawn srv;
srv.request.x = 0.5 + (rand()%100) / 10;
srv.request.y = 0.5 + (rand()%100) / 10 ;
srv.request.theta = rand()%360;
//Input the name in command line and transfer to request
std::string name;
ROS_INFO("Please input the turtle name: ");
std::getline(std::cin, name);
srv.request.name = name;
if (client.call(srv)){
ROS_INFO("Response: [%s]", srv.request.name.c_str());
}else{
ROS_INFO("Failed to create a turtle!");
}
return 0;
}<file_sep>/assignment1/src/turtle_spawn_server.cpp
#include <ros/ros.h>
#include <assignment1/turtlecall.h>
#include <turtlesim/Spawn.h>
#include <ctime>
class turtlecall{
public:
turtlecall(){
client = n.serviceClient<turtlesim::Spawn>("/spawn");
service= n.advertiseService("/turtlecall", &turtlecall::Callback,this);
}
bool Callback(assignment1::turtlecall::Request &req, assignment1::turtlecall::Response &res){
srand((unsigned int)time(NULL));
turtlesim::Spawn srv;
srv.request.x = 0.5 + (rand()%100)/10;
srv.request.y = 0.5 + (rand()%100)/10;
srv.request.theta = rand()%360;
srv.request.name = req.name;
if (client.call(srv)){
ROS_INFO("Successfully call a turtle!");
res.result = "Success!";
}else{
res.result = "Wrong!";
}
return true;
}
private:
ros::NodeHandle n;
ros::ServiceClient client;
ros::ServiceServer service;
};
int main(int argc, char **argv){
ros::init(argc, argv, "turtlecall_service");
ros::service::waitForService("/spawn");
ROS_INFO("Ready to call the turtle");
turtlecall A;
ros::spin();
return 0;
}<file_sep>/assignment1/src/turtle_spawn_client.cpp
#include <ros/ros.h>
#include <turtlesim/Spawn.h>
#include <assignment1/turtlecall.h>
#include<iostream>
int main(int argc, char **argv){
ros::init(argc, argv, "turtlecall_client");
ros::NodeHandle n;
ros::service::waitForService("/turtlecall");
ros::ServiceClient client = n.serviceClient<assignment1::turtlecall>("/turtlecall");
assignment1::turtlecall srv;
std::string name;
ROS_INFO("Please input the turtle name:");
std::getline(std::cin,name);
srv.request.name = name;
ROS_INFO("Call service to add a turtle [%s]",srv.request.name.c_str());
client.call(srv);
ROS_INFO("Show response: [%s]",srv.response.result.c_str());
return 0;
}<file_sep>/assignment1/src/turtle_control.cpp
#include <ros/ros.h>
#include <geometry_msgs/Twist.h>
#include <iostream>
int main(int argc, char **argv){
ros::init(argc, argv, "turtle_control");
ros::NodeHandle n;
ROS_INFO("Please input the target turtle:");
std::string name;
std::getline(std::cin, name);
ros::Publisher pub = n.advertise<geometry_msgs::Twist>("/"+name+"/cmd_vel",1);
ros::Rate loop_rate(10);
ROS_INFO("Please input the angular velocity of turtle:");
double w;
std::cin>>w;
ROS_INFO("Please input the linear velocity of turtle:");
double x;
std::cin>>x;
geometry_msgs::Twist msg;
msg.linear.x = (double)x;
msg.linear.y = 0;
msg.linear.z = 0;
msg.angular.x = 0;
msg.angular.y = 0;
msg.angular.z = (double)w;
while (ros::ok()){
pub.publish(msg);
loop_rate.sleep();
}
return 0;
} | c5feefe425059aa7df24f095a8b04c3143f67c17 | [
"Markdown",
"C++"
] | 7 | C++ | Kyle-Xu001/ROS-Practice | 6c2cc550dcdf408828896427daa65d91c80f25d6 | ced976c08bd2a6aa3343b35b68eda7994248fb23 | |
refs/heads/main | <file_sep>package com.leanmentors.goals
import com.leanmentors.goals.controllers.TaskController
import com.leanmentors.goals.data.Tasks
import org.junit.jupiter.api.Test
import reactor.test.StepVerifier
import java.time.LocalDate
class TaskServiceTest {
@Test
fun testController() {
StepVerifier.create(TaskController().createTasks())
.expectNext(Tasks("Create Project", "Create Project Today", LocalDate.parse("2020-10-05"), LocalDate.parse("2020-10-05")))
.verifyComplete()
}
}<file_sep>package com.leanmentors.goals
import com.leanmentors.goals.controllers.TaskController
import com.leanmentors.goals.data.Tasks
import io.micronaut.http.HttpRequest
import io.micronaut.http.HttpResponse
import io.micronaut.http.client.annotation.Client
import io.micronaut.reactor.http.client.ReactorHttpClient
import io.micronaut.runtime.server.EmbeddedServer
import io.micronaut.test.extensions.junit5.annotation.MicronautTest
import org.junit.jupiter.api.Assertions.assertEquals
import org.junit.jupiter.api.Test
import reactor.core.publisher.Flux
import reactor.test.StepVerifier
import java.util.*
import javax.inject.Inject
import kotlin.reflect.jvm.internal.impl.metadata.ProtoBuf
@MicronautTest
class TaskServiceIntTest {
@Inject
lateinit var server: EmbeddedServer
@Inject
@field:Client("/")
lateinit var client: ReactorHttpClient
@Test
fun testHelloWorldResponse() {
val response: Flux<HttpResponse<Tasks>>? = client.exchange("/tasks", Tasks::class.java)
response?.map { it.body() }?.count()
}
}
<file_sep>plugins {
id "org.jetbrains.kotlin.jvm" version "1.4.10"
id "org.jetbrains.kotlin.kapt" version "1.4.10"
id "org.jetbrains.kotlin.plugin.allopen" version "1.4.10"
id "com.github.johnrengelman.shadow" version "6.0.0"
id "io.micronaut.application" version '1.0.5'
}
version "0.1"
group "com.example"
repositories {
mavenCentral()
jcenter()
}
micronaut {
runtime "netty"
testRuntime "junit5"
processing {
incremental true
annotations "com.example.*"
}
}
dependencies {
implementation("io.micronaut:micronaut-validation")
implementation("org.jetbrains.kotlin:kotlin-stdlib-jdk8:${kotlinVersion}")
implementation("org.jetbrains.kotlin:kotlin-reflect:${kotlinVersion}")
implementation("io.micronaut.kotlin:micronaut-kotlin-runtime")
implementation("io.micronaut:micronaut-runtime")
implementation("io.micronaut:micronaut-http-client")
implementation("io.micronaut.netflix:micronaut-netflix-hystrix")
implementation("io.micronaut.beanvalidation:micronaut-hibernate-validator")
implementation("io.micronaut.mongodb:micronaut-mongo-reactive")
implementation("io.micronaut.reactor:micronaut-reactor")
runtimeOnly("ch.qos.logback:logback-classic")
runtimeOnly("com.fasterxml.jackson.module:jackson-module-kotlin")
annotationProcessor("io.micronaut:micronaut-inject-java:2.1.3") // <2>
implementation("io.micronaut:micronaut-inject:2.1.3")
testAnnotationProcessor("io.micronaut:micronaut-inject-java:2.1.3")
testImplementation("de.flapdoodle.embed:de.flapdoodle.embed.mongo:2.0.1")
testImplementation("io.micronaut.reactor:micronaut-reactor-http-client:1.0.0")
testImplementation("io.projectreactor:reactor-test:3.1.0.RELEASE")
}
mainClassName = "com.example.ApplicationKt"
java {
sourceCompatibility = JavaVersion.toVersion('1.8')
}
compileKotlin {
kotlinOptions {
jvmTarget = '1.8'
}
}
compileTestKotlin {
kotlinOptions {
jvmTarget = '1.8'
}
}
<file_sep>package com.leanmentors.goals.data
import java.time.LocalDate
data class Tasks(val name: String,
val description: String,
val startDate: LocalDate,
val endDate: LocalDate)<file_sep>package com.leanmentors.goals.controllers
import com.leanmentors.goals.data.Tasks
import io.micronaut.http.MediaType
import io.micronaut.http.annotation.Controller
import io.micronaut.http.annotation.Get
import reactor.core.publisher.Flux
import java.time.LocalDate
import java.util.*
@Controller("/tasks")
class TaskController {
@Get(produces = [MediaType.APPLICATION_JSON] )
fun createTasks():Flux<Tasks>{
return Flux.just(Tasks("Create Project", "Create Project Today", LocalDate.parse("2020-10-05"), LocalDate.parse("2020-10-05")))
}
} | 4be82ee630b21ffc61103fd53fb9e5ef3b56b40e | [
"Kotlin",
"Gradle"
] | 5 | Kotlin | khurramsyed/task-service | 13c03f5cf7c34c14294e6bfba63a61083a301582 | 2e184c05e45ff3a339324667c7b3e0f3e1feae2f | |
refs/heads/master | <file_sep>using System;
using System.Collections.Generic;
using System.Diagnostics.SymbolStore;
using System.Linq;
using System.Net.NetworkInformation;
using System.Threading.Tasks;
namespace C_Sharp_Challenge_Skeleton.Answers
{
public class Question1
{
public static int Answer(int[] portfolios)
{
var maxValue = 0;
for (var i = 0; i < portfolios.Length; i++)
{
for (var j = 0; j < portfolios.Length; j++)
{
int xor = portfolios[i] ^ portfolios[j];
if (xor > maxValue)
{
maxValue = xor;
}
}
}
return maxValue;
}
}
}
<file_sep>using System;
using System.Linq;
namespace C_Sharp_Challenge_Skeleton.Answers
{
public class Question5
{
public static int Answer(int[] numOfShares, int totalValueOfShares)
{
var numOfShares2 = numOfShares.ToList();
int count = 1;
while (calSets(totalValueOfShares, numOfShares2.ToArray()) == 0)
{
for (int i = 0; i < numOfShares.Length; i++)
{
numOfShares2.Add(numOfShares[i]);
}
count++;
}
return count;
}
static int[,] costs;
static int[,] minItems;
public static int calSets(int target, int[] arr) {
costs = new int[arr.Length, target + 1];
minItems = new int[arr.Length, target + 1];
for (int j = 0; (j <= target); j++) {
if ((arr[0] <= j)) {
costs[0, j] = arr[0];
minItems[0, j] = 1;
}
}
for (int i = 1; (i < arr.Length); i++) {
for (int j = 0; (j <= target); j++) {
costs[i, j] = costs[(i - 1), j];
minItems[i, j] = minItems[(i - 1), j];
if ((arr[i] <= j)) {
costs[i, j] = Math.Max(costs[i, j], (costs[(i - 1), (j - arr[i])] + arr[i]));
if ((costs[(i - 1), j]
== (costs[(i - 1), (j - arr[i])] + arr[i]))) {
minItems[i, j] = Math.Min(minItems[i, j], (minItems[(i - 1), (j - arr[i])] + 1));
}
else if ((costs[(i - 1), j]
< (costs[(i - 1), (j - arr[i])] + arr[i]))) {
minItems[i, j] = (minItems[(i - 1), (j - arr[i])] + 1);
}
}
}
}
if ((costs[(arr.Length - 1), target] == target))
{
return minItems[(arr.Length - 1), target];
}
return 0;
}
}
}<file_sep>using System.Collections.Generic;
using System.Linq;
namespace C_Sharp_Challenge_Skeleton.Answers
{
public class Question4
{
public static int Answer(string[,] machineToBeFixed, int numOfConsecutiveMachines)
{
int dim = machineToBeFixed.GetLength(0);
int max = 999;
for (int i = 0; i < dim; i++)
{
var row = SliceRow(machineToBeFixed, i);
if (dim - row.Count(x => x == "X") == numOfConsecutiveMachines)
{
List<string> rowString = row.ToList();
rowString.RemoveAll(x => x == "X");
List<int> rowInt = rowString.Select(int.Parse).ToList();
if (rowInt.Sum() < max)
{
max = rowInt.Sum();
}
}
}
if (max == 999) max = 0;
return max;
}
public static IEnumerable<T> SliceRow<T>(T[,] array, int row)
{
for (var i = array.GetLowerBound(1); i <= array.GetUpperBound(1); i++)
{
yield return array[row, i];
}
}
}
}<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using C_Sharp_Challenge_Skeleton.Beans;
using QuickGraph;
namespace C_Sharp_Challenge_Skeleton.Answers
{
public class Question3
{
public static int Answer(int numOfNodes, Edge[] edgeLists)
{
List<AdjacencyGraph<int, Edge<int>>> graphs = new List<AdjacencyGraph<int, Edge<int>>>();
List<List<int>> possibleSolutions = new List<List<int>>();
List<int> nodes = Enumerable.Range(1, numOfNodes).ToList();
int comboCount = (int) Math.Pow(2, nodes.Count) - 1;
List<List<int>> combos = new List<List<int>>();
for (int i = 1; i < comboCount + 1; i++)
{
combos.Add(new List<int>());
for (int j = 0; j < nodes.Count; j++)
{
if ((i >> j) % 2 != 0)
combos.Last().Add(nodes[j]);
}
}
for (var index = 0; index < combos.Count; index++)
{
var combo = combos[index];
AdjacencyGraph<int, Edge<int>> graph = new AdjacencyGraph<int, Edge<int>>();
for (var index1 = 0; index1 < combo.Count; index1++)
{
var i = combo[index1];
graph.AddVertex(i);
}
bool dontAdd = false;
for (var i = 0; i < edgeLists.Length; i++)
{
var e = edgeLists[i];
if (graph.ContainsVertex(e.EdgeA) && graph.ContainsVertex(e.EdgeB))
{
dontAdd = true;
}
}
if (!dontAdd)
{
graphs.Add(graph);
}
}
AdjacencyGraph<int, Edge<int>> selectedGraph = new AdjacencyGraph<int, Edge<int>>();
foreach (var graph in graphs.ToList())
{
if (graph.Edges.Any() || graph.VertexCount == 1)
{
graphs.Remove(graph);
}
else
{
if (graph.VertexCount > selectedGraph.VertexCount) selectedGraph = graph;
}
}
int X = selectedGraph.VertexCount;
int Y = numOfNodes - X;
return X - Y;
}
}
}<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace C_Sharp_Challenge_Skeleton.Answers
{
public class Question2
{
public static int Answer(int[] cashflowIn, int[] cashflowOut)
{
List<IEnumerable<int>> inSets = Subsets(cashflowIn.ToList()).Where(x => x.Count() != 0).ToList();
List<IEnumerable<int>> outSets = Subsets(cashflowOut.ToList()).Where(x => x.Count() != 0).ToList();
int minDiff = Math.Abs(inSets.ElementAt(0).Sum() - outSets.ElementAt(0).Sum());
foreach (var set in inSets)
{
foreach (var set2 in outSets)
{
if (Math.Abs(set.Sum() - set2.Sum()) < minDiff)
{
minDiff = Math.Abs(set.Sum() - set2.Sum());
}
}
}
return minDiff;
}
public static IEnumerable<IEnumerable<T>> Subsets<T>(IEnumerable<T> source)
{
List<T> list = source.ToList();
int length = list.Count;
int max = (int)Math.Pow(2, list.Count);
for (int count = 0; count < max; count++)
{
List<T> subset = new List<T>();
uint rs = 0;
while (rs < length)
{
if ((count & (1u << (int)rs)) > 0)
{
subset.Add(list[(int)rs]);
}
rs++;
}
yield return subset;
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using QuickGraph;
using QuickGraph.Algorithms;
namespace C_Sharp_Challenge_Skeleton.Answers
{
public class Question6
{
static AdjacencyGraph<int, Edge<int>> graph = new AdjacencyGraph<int, Edge<int>>();
static Dictionary<Edge<int>, double> costs = new Dictionary<Edge<int>, double>();
public static int Answer(int numOfServers, int targetServer, int[,] connectionTimeMatrix)
{
for (int i = 0; i < connectionTimeMatrix.GetLength(0); i++)
{
for (int j = 0; j < numOfServers; j++)
{
var edge = new Edge<int>(i, j);
graph.AddVerticesAndEdge(edge);
costs.Add(edge, connectionTimeMatrix[i,j]);
}
}
var edgeCost = AlgorithmExtensions.GetIndexer(costs);
var tryGetPath = graph.ShortestPathsDijkstra(edgeCost, 0);
IEnumerable<Edge<int>> path;
tryGetPath(targetServer, out path);
double cost = 0;
foreach (var edge in path)
{
cost += costs[edge];
}
return Convert.ToInt32(cost);
}
}
}
| 185827125a17e5de0d5745d7651fd0f0e9631ea2 | [
"C#"
] | 6 | C# | ashmcmn/csharp-skeleton | aec0b15bf67f1d9995bebb992dc008db14d8806f | 840004c3ab3bdd1fdeb8db8913c6a81b9cbac273 | |
refs/heads/master | <file_sep>
document.getElementById('search-btn').addEventListener('click',function(){
const searchBar = document.getElementById('search-bar').value;
fetch (`https://api.lyrics.ovh/suggest/${searchBar}`)
.then(res=>res.json())
.then ( data =>{
// console.log(data.data.id)
data= data.data.slice(0,10);
const listArea = document.getElementById('show-area');
listArea.innerHTML='';
for (let i = 0; i < data.length; i++) {
const songs = data[i];
// console.log(songs)
// searchBar = '';
const p = document.createElement('p');
p.innerHTML= `
<div class="single-result row align-items-center my-3 p-3">
<div class="col-md-9">
<h3 class="lyrics-name">${songs.title}</h3>
<p class="author lead">Album by <span>${songs.artist.name}</span></p>
</div>
<div class="col-md-3 text-md-right text-center">
<button class="btn btn-success">Get Lyrics</button>
</div>
</div>
`
listArea.appendChild(p);
}
})
})
| a0979b9b35c7b9ff240d12d6be47ae256719f670 | [
"JavaScript"
] | 1 | JavaScript | MdGolamJakaria/hard-rock-master | 028e0a29ccefd7d02485dd8e97800758109eed56 | f0abd2cab2761c2da1adffeada11d4c76ae056d1 | |
refs/heads/master | <repo_name>kaikuenne/rails-task-manager<file_sep>/config/routes.rb
Rails.application.routes.draw do
# For details on the DSL available within this file, see http://guides.rubyonrails.org/routing.html
#index all tasks
get 'tasks', to: 'tasks#index', as: :index
#show one task
get 'tasks/:id', to: 'tasks#show', as: :task
#create a task
get 'new', to: 'tasks#new', as: :new_task
post 'tasks', to: 'tasks#create'
#update a task
get 'tasks/:id/edit', to: 'tasks#edit', as: :edit_task
patch 'tasks/:id', to: 'tasks#update'
#delete a task
delete 'tasks/:id', to: 'tasks#destroy', as: :delete_task
end
| e46d61243bba754d5e99c21c84953a5127909d7d | [
"Ruby"
] | 1 | Ruby | kaikuenne/rails-task-manager | ddb67987184a69a1cac3b2695792fce49a8aca9a | c59aad88019d902f8a5621d70d139f4dad122115 | |
refs/heads/master | <repo_name>jesscjess/TDD-final-project<file_sep>/test/routes/create-video-test.js
// Step 1 create the default files for this level for testing
// Step 2 have examples show what you can do with app to make simple tests
//
// Can explain this in docs
//
// Create easy way to add tests that provide specific information
//
// should we look into chai-webdriver or is chai enough??
const { assert } = require('chai');
const request = require('supertest');
const { jsdom } = require('jsdom');
const app = require('../../app');
const Video = require('../../models/video');
const { parseTextFromHTML, buildItemObject } = require('../test-helper-funcs');
const { connectDatabase, disconnectDatabase } = require('../db-helper-funcs');
describe('Server path: /videos/create ->', () => {
beforeEach(connectDatabase);
afterEach(disconnectDatabase);
describe('POST ->', () => {
it('create new video', async () => {
const newVideo = buildItemObject();
const response = await request(app)
.post('/videos/create')
.type('form')
.send(newVideo);
const createdItem = await Video.findOne(newVideo);
assert.equal(response.status, 302);
assert.isOk(createdItem, 'Looks like item was not created successfully in the database');
});
it('cannot create video with NO title', async () => {
const newVideo = buildItemObject();
newVideo.title = '';
const response = await request(app)
.post('/videos/create')
.type('form')
.send(newVideo);
assert.deepEqual(await Video.find({}), []);
assert.equal(response.status, 400);
assert.include(parseTextFromHTML(response.text, 'form'), 'Path `title` is required.');
assert.include(parseTextFromHTML(response.text, 'form'), newVideo.description);
});
});
});
<file_sep>/test/features/user-visits-create-page-test.js
const {assert} = require('chai');
const {buildItemObject} = require('../test-helper-funcs')
describe('User visits create page ->', () => {
describe('creates new video ->', () => {
it('sees new video on main page', () => {
browser.url('/videos/create');
const newItem = buildItemObject()
browser.setValue('#title-input', newItem.title);
browser.setValue('#description-input', newItem.description);
browser.click('#submit-button')
assert.include(browser.getText('body'), newItem.title);
assert.include(browser.getText('body'), newItem.description);
});
});
});<file_sep>/test/test-helper-funcs.js
const {jsdom} = require('jsdom');
const Video = require('../models/video');
const buildItemObject = (options = {}) => {
const title = options.title || 'New video I made up';
const description = options.description || 'This is a video I made up durrr';
const url = options.url || 'https://www.youtube.com/embed/UcfOIfa5kvE';
return {title, description, url};
};
// Add a sample Item object to mongodb
const seedItemToDatabase = async (options = {}) => {
const item = await Video.create(buildItemObject(options));
return item;
};
// extract text from an Element by selector.
const parseTextFromHTML = (htmlAsString, selector) => {
const selectedElement = jsdom(htmlAsString).querySelector(selector);
if (selectedElement !== null) {
return selectedElement.textContent;
} else {
throw new Error(`No element with selector ${selector} found in HTML string`);
}
};
module.exports = {
buildItemObject,
seedItemToDatabase,
parseTextFromHTML
};<file_sep>/test/models/video-test.js
const Video = require('../../models/video')
const { assert } = require('chai');
const { mongoose, databaseUrl, options } = require('../../database');
const {connectDatabase, disconnectDatabase} = require('../db-helper-funcs');
describe('Video Model ->', () => {
beforeEach(connectDatabase);
afterEach(disconnectDatabase);
describe('#Title ->', () => {
it('is a String', () => {
const integerTitle = 9;
const video = new Video({ title: integerTitle });
assert.strictEqual(video.title, integerTitle.toString());
})
});
describe('#Description ->', () => {
it('is a String', () => {
const integerDescription = 9;
const video = new Video({ description: integerDescription });
assert.strictEqual(video.description, integerDescription.toString());
})
});
// Question: is it better to not test if something is required here so that I can test it with the error message ar the routes level?
});
<file_sep>/test/features/user-visits-landing-page-test.js
const {assert} = require('chai');
const { parseTextFromHTML, seedItemToDatabase, buildItemObject } = require('../test-helper-funcs');
describe('User hits main page ->', () => {
describe('with no videos added yet ->', () => {
it('page has no items', () => {
browser.url('/videos');
assert.equal(browser.getText('#videos-container'), '')
});
});
describe('with existing videos ->', () => {
it('page shows iframe', () => {
browser.url('/videos/create');
const newVideo = buildItemObject();
browser.setValue('#title-input', newVideo.title);
browser.setValue('#description-input', newVideo.description);
browser.setValue('#url-input', newVideo.url);
browser.url('/');
assert.include(browser.getText('body'), 'iframe');
});
});
describe('can navigate ->', () => {
it('to videos/create', () => {
browser.url('/videos');
// browser.click('a[href="/videos/create]') -> not wokring :/
browser.click('a')
assert.include(browser.getText('body'), 'Add Video');
});
});
});
<file_sep>/test/routes/single-video-test.js
const { assert } = require('chai');
const request = require('supertest');
const app = require('../../app');
const { parseTextFromHTML, seedItemToDatabase } = require('../test-helper-funcs');
const { connectDatabase, disconnectDatabase } = require('../db-helper-funcs');
describe('Server path: /video/:id', () => {
beforeEach(connectDatabase);
afterEach(disconnectDatabase);
// Write your test blocks below:
describe('GET', () => {
it('correct video rendered', async () => {
const newVideo = await seedItemToDatabase();
const videoUrl = '/video/' + newVideo._id.toString();
const response = await request(app)
.get(videoUrl);
assert.equal(response.status, 200);
assert.include(parseTextFromHTML(response.text, '#item-title'), newVideo.title);
assert.include(parseTextFromHTML(response.text, '#item-description'), newVideo.description);
})
})
});<file_sep>/README.md
# TDD-final-project
| fc0d2268683499fa5cdc9db93bb169d9fedee3fb | [
"JavaScript",
"Markdown"
] | 7 | JavaScript | jesscjess/TDD-final-project | 9d471749f28407a1160b1a3b22c9a96405297605 | 0ab131183208f8193503555ad671989fe16fede2 | |
refs/heads/master | <repo_name>cj814/YiKaTong<file_sep>/src/mock/caipu.js
// 引入mockjs
const Mock = require('mockjs');
// 获取 mock.Random 对象
const Random = Mock.Random;
// 菜谱列表
const caiPuList = () => {
let caiPuList = [];
for(let i = 0; i < 10; i++) {
caiPuList.push({
caiPuName: Random.csentence(4, 6),
caiPuImage: Random.dataImage('100%x100%', '随机菜谱')
})
}
return caiPuList
}
// 菜谱详情
const caiPuDetail = () => {
let caiPuDetail = {
danBaiZhi: Random.float(0, 100, 2, 2) + '%',
xianWeiSu: Random.float(0, 100, 2, 2) + '%',
huaHeWu: Random.float(0, 100, 2, 2) + '%',
caiPuScore: Random.integer(0, 5),
caiPuName: Random.csentence(4, 6),
caiPuImage: Random.dataImage('100%x100%', '随机菜谱')
}
return caiPuDetail
}
export default {
getCaiPuList: caiPuList,
getCaiPuDetail: caiPuDetail
}<file_sep>/src/mock/account.js
// 引入mockjs
const Mock = require('mockjs');
// 获取 mock.Random 对象
const Random = Mock.Random;
// 账户信息
const accountInfo = () => {
let accountInfo = {
userName: Random.cname(),
cardNumber: Random.natural(),
bankName: Random.csentence(4, 6),
bankLogo: Random.dataImage('100%x100%', 'B')
}
return accountInfo
}
export default {
getAccountInfo: accountInfo
}<file_sep>/src/mock/cost.js
// 引入mockjs
const Mock = require('mockjs');
// 获取 mock.Random 对象
const Random = Mock.Random;
// 消费列表
const costList = () => {
let costList = [];
for (let i = 0; i < 10; i++) {
costList.push({
costName: Random.csentence(6, 8),
costTime: Random.datetime('MM-dd HH:mm'),
costMoney: Random.float(0, 1000, 2, 2)
})
}
return costList
}
// 消费详情
const costDetail = () => {
let costDetail = {
costName: Random.csentence(6, 8),
yingYeShiDuan: Random.csentence(2, 4),
costMoney: Random.float(0, 1000, 2, 2),
qingCaiBao: Random.float(0, 1000, 2, 2),
kaNeiJinE: Random.float(0, 1000, 2, 2),
costTime: Random.datetime('yyyy-MM-dd HH:mm')
};
return costDetail
}
export default {
getCostList: costList,
getCostDetail: costDetail
}<file_sep>/src/mock/kaoqin.js
// 引入mockjs
const Mock = require('mockjs');
// 获取 mock.Random 对象
const Random = Mock.Random;
// 考勤状态
const kaoQinState = () => {
let kaoQinState = {
chuQinTianShu: Random.integer(1, 31),
xiuXiTianShu: Random.integer(1, 31),
chiDao: Random.integer(1, 31),
zaoTui: Random.integer(1, 31),
kuangGong: Random.integer(1, 31),
jiaBan: Random.integer(1, 31)
}
return kaoQinState
}
// 考勤详情
const kaoQinDetail = () => {
let kaoQinDetail = {
shangBanTime: Random.datetime('HH:mm'),
xiaBanTime: Random.datetime('HH:mm')
}
return kaoQinDetail
}
export default {
getUserKaoQinState: kaoQinState,
getUserKaoQinDetail: kaoQinDetail
}<file_sep>/src/api/cost.js
import { fetch } from '../utils/fetch'
// 消费列表
export function getCostList(url, params) {
return fetch(url, params)
}
// 消费详情
export function getCostDetail(url, params) {
return fetch(url, params)
}<file_sep>/src/api/kaoqin.js
import { fetch } from '../utils/fetch'
// 考勤状态
export function getUserKaoQinState(url, params) {
return fetch(url, params)
}
// 考勤详情
export function getUserKaoQinDetail(url, params) {
return fetch(url, params)
}<file_sep>/src/router/index.js
import Vue from 'vue'
import Router from 'vue-router'
import App from '../App'
import Layout from '../views/layout'
import kaoQinRecord from '../views/kaoQinRecord'
import costRecord from '../views/costRecord'
import oneWeekMenu from '../views/oneWeekMenu'
import menuDetail from '../views/menuDetail'
import costDetail from '../views/costDetail'
import foodEvaluate from '../views/foodEvaluate'
import myAccount from '../views/myAccount'
import transMoney from '../views/transMoney'
import kaoQinDate from '../views/kaoQinDate'
import kaoQinDetail from '../views/kaoQinDetail'
Vue.use(Router)
export default new Router({
routes: [
{
path: '/',
name: 'Layout',
component: Layout
},
{
path: '/views/kaoQinRecord',
name: 'kaoQinRecord',
component: kaoQinRecord
},
{
path: '/views/kaoQinDetail',
name: 'kaoQinDetail',
component: kaoQinDetail
},
{
path: '/views/oneWeekMenu',
name: 'oneWeekMenu',
component: oneWeekMenu
},
{
path: '/views/costRecord',
name: 'costRecord',
component: costRecord
},
{
path: '/views/menuDetail',
name: 'menuDetail',
component: menuDetail
},
{
path: '/views/costDetail',
name: 'costDetail',
component: costDetail
},
{
path: '/views/foodEvaluate',
name: 'foodEvaluate',
component: foodEvaluate
},
{
path: '/views/myAccount',
name: 'myAccount',
component: myAccount
},
{
path: '/views/transMoney',
name: 'transMoney',
component: transMoney
},
{
path: '/views/kaoQinDate',
name: 'kaoQinDate',
component: kaoQinDate
}
]
})
<file_sep>/README.md
# 一卡通考勤(公司vue-cli项目demo)
## 安装
``` bash
# 安装项目依赖
npm install
# 运行
npm run dev
# 编译
npm run build
```
在线预览:https://cj814.github.io/YiKaTong/docs/ | 4f77de5b02223c7a92195389ea62e8cbc852a516 | [
"JavaScript",
"Markdown"
] | 8 | JavaScript | cj814/YiKaTong | 7b4b8962b4f0e93d5b05724bacd9a40a7956f60c | c06f835cac8b46264aef9601adcb732b0b75406c | |
refs/heads/master | <repo_name>ielgstrom/C2-ejercio-5<file_sep>/js/script.js
const palindromo = "Àl!imüUmîlä";
const noPalindromo = "tengoUnGato";
function esPalindromo(string) {
let cambio = string;
const esPalindoromo = false;
cambio = cambio.toLowerCase();
cambio = cambio.replace(/[.,:;()_?¿!¡-\s]/g, "");
cambio = cambio.normalize("NFD").replace(/[\u0300-\u036f]/g, "");
const cambioArray = cambio.split("");
const cambioReverseArray = cambioArray.reverse();
const cambioReverse = cambioReverseArray.join("");
console.log(
`${cambioReverse}${
cambioReverse === cambio ? "" : " no"
} es palindorm ja que l'inversa es ${cambio}`
);
}
esPalindromo(palindromo);
esPalindromo(noPalindromo);
| 384100635fe7d43ca8db9240a8529d490c80b06f | [
"JavaScript"
] | 1 | JavaScript | ielgstrom/C2-ejercio-5 | 2e172ae128ab4995eb198317688423e6f52b1dca | bd2991f26070aa09c76e8aa240827bc999c44204 | |
refs/heads/master | <file_sep>class CarOwner
attr_reader :name
@@all = []
def initialize(name)
@name = name
@@all << self
end
def self.all
@@all
end
def cars
Car.all.select{|car| car.owner == self}
end
def mechanics
# car_class = cars.map{|car| car.classification}
cars.map{|car| car.mechanic}
end
def self.average
Car.all.count/all.count
end
end
<file_sep>require_relative '../app/models/mechanic.rb'
require_relative '../app/models/car.rb'
require_relative '../app/models/car_owner.rb'
require_relative '../config/environment.rb'
require 'pry'
def reload
load 'config/environment.rb'
end
co1 = CarOwner.new("Aaron")
co2 = CarOwner.new("Ed")
co3 = CarOwner.new("Scott")
mech1 = Mechanic.new("Danielle", "small car")
mech2 = Mechanic.new("Flatiron", "sports car")
mech3 = Mechanic.new("Will", "classic car")
car1 = Car.new("Mini", "Clubman", "small car", mech1, co1)
car2 = Car.new("Ferrari", "California", "sports car", mech2, co3)
car3 = Car.new("Ferrari", "<NAME>", "sports car", mech2, co2)
car4 = Car.new("Datsun", "280z", "classic car", mech3, co2)
binding.pry
0<file_sep>class Mechanic
attr_reader :name, :specialty
@@all = []
def initialize(name, specialty)
@name = name
@specialty = specialty
@@all << self
end
def self.all
@@all
end
def cars
Car.all.select{|car| car.mechanic == self}
end
def car_owners
cars.map{|car| car.owner}.uniq
end
def car_owner_names
car_owners.map{|owner| owner.name}
end
end
| 182c7b5acb99bfe7a68d1274f23599440b3eecbe | [
"Ruby"
] | 3 | Ruby | EJWebber/OO-Auto-Shop-london-web-060319 | 54472097fa15df835807229333ea85ff933e5d8b | 998ad738098e4da3658da8f399488283bdff9c28 | |
refs/heads/master | <file_sep>//
// Projectile.swift
// D&Doom
//
// Created by <NAME> on 2016-02-18.
// Copyright © 2016 <NAME>. All rights reserved.
//
import Foundation
import UIKit
import GLKit
import OpenGLES
class Projectile : Actor {
var homeInOnPlayer = false;
var damage = 20; //to be set manually
//x and y are mouse screen coordinates, and z is the depth that this will have, for the current projection and modelview
init() {
super.init(position: ActorConstants._origin);
}
init(screenX:CGFloat, screenY:CGFloat, farplaneZ:Int, speed:Float, modelView:GLKMatrix4, projection:GLKMatrix4, viewport:UnsafeMutablePointer<Int32>, mVars:modelVars) {
let _vecDest = GLKMathUnproject(GLKVector3Make(Float(screenX), Float(screenY), Float(farplaneZ)), modelView, projection, viewport, nil);
let _vecOrigin = GLKMathUnproject(Actor.ActorConstants._origin, modelView, projection, viewport, nil);
super.init(position: ActorConstants._origin);
let lineVectorWorld = GLKVector3Subtract(_vecDest, _vecOrigin);
//var success : UnsafeMutablePointer<Bool> -> GLKVector3;
//var success = nil;
//z would be the far FOV
_position = ActorConstants._origin;
//Only normalize if it's not 0 - otherwise it would return nil (and it multiplying speed by 0 won't change the value anyway)
print("args: (\(screenX), \(screenY), \(farplaneZ))");
printVector("vecDest: ", vec: lineVectorWorld);
printVector("lineVectorWorld: ", vec: lineVectorWorld);
if(GLKVector3Length(lineVectorWorld) != 0) {
_velocity = GLKVector3MultiplyScalar(GLKVector3Normalize(lineVectorWorld), speed);
}
printVector("velocity: ", vec: _velocity);
}
init(position:GLKVector3, velocity:GLKVector3, mVars:modelVars) {
super.init(position: ActorConstants._origin);
_velocity = velocity;
_modelVars = mVars;
}
func setModel(mVars:modelVars) {
//copy the struct
_modelVars = mVars;
}
//For debug purposes.
func printVector(label : String, vec : GLKVector3) {
print("\(label): (\(vec.x), \(vec.y), \(vec.z))");
}
func printVector(vec : GLKVector3) {
print("(\(vec.x), \(vec.y), \(vec.z))");
}
//Update projectile position and velocity. Check collisions.
override func update() {
//Get amount per frame, rather than per second
let accel_f = GLKVector3DivideScalar(_acceleration, 60);
_velocity = GLKVector3Add(_velocity, accel_f);
let velocity_f = GLKVector3DivideScalar(_velocity, 60); _position = GLKVector3Add(_position, velocity_f);
//print("\(_position.x), \(_position.y), \(_position.z)");
if(homeInOnPlayer) {
homingOn(GameViewController.position);
}
checkCollision();
//printVector("Position: ", vec: _position);
}
func checkCollision() {
//check z coordinate matching any other enemies
//then do a 2D collision detection ...
//though that may involve using a buffer ...
var collided = false;
var other : Actor;
//Or, just can do the very efficient and simple bounding spheres collision.
//Not sure how to handle model data for other forms of collision at the moment.
for var ActorList in GameViewController.ActorLists { //if var not specified, "let" is default
for (var i=0; i<ActorList.count; i++) {
let actor = ActorList[i]; //force downcast/unwrap with "as!"
if(GLKVector3Length(GLKVector3Subtract(_position, actor._position)) <= Float(actor._radius + _radius)) {
collided = true;
other = actor;
//handle collision code here, in order to preserve the index of (and thus ability to remove) ActorList elements
//destroy enemy, damage player, depending on what kind of projectile it is. Could use homeInOnPlayer if and only if the projectiles are enemy projectiles targeting the player, perhaps. Given that:
if(homeInOnPlayer) {
//damage player
GameViewController.mHealth -= damage;
}
else {
//Checking enemy - kill enemy if it's one
//Could make it damage the enemy instead
if let enemy = ActorList[i] as? Enemy {
enemy._health -= damage;
if(enemy._health <= 0) {
//Remove enemy as the kill process.
ActorList.removeAtIndex(i);
}
}
}
break;
}
}
}
}
//Credit goes to http://stackoverflow.com/questions/15247347/collision-detection-between-a-boundingbox-and-a-sphere-in-libgdx
//Sphere and bounding box (AABB?) collision
/*
public static boolean sphereBBCollides(BoxmVars : modelVars) {
float dmin = 0;
Vector3 center = sphere.center;
Vector3 bmin = boundingBox.getMin();
Vector3 bmax = boundingBox.getMax();
if (center.x < bmin.x) {
dmin += Math.pow(center.x - bmin.x, 2);
} else if (center.x > bmax.x) {
dmin += Math.pow(center.x - bmax.x, 2);
}
if (center.y < bmin.y) {
dmin += Math.pow(center.y - bmin.y, 2);
} else if (center.y > bmax.y) {
dmin += Math.pow(center.y - bmax.y, 2);
}
if (center.z < bmin.z) {
dmin += Math.pow(center.z - bmin.z, 2);
} else if (center.z > bmax.z) {
dmin += Math.pow(center.z - bmax.z, 2);
}
return dmin <= Math.pow(sphere.radius, 2);
}*/
//Home in on target
func homingOn(target:GLKVector3) {
var vecDir = GLKVector3Subtract(target, self._position);
vecDir = GLKVector3Normalize(vecDir); //unit vector used to get direction of vector
var projVelocity = GLKVector3MultiplyScalar(vecDir, GLKVector3Length(_velocity))
_velocity = projVelocity;
}
}<file_sep>//
// PlayerData.swift
// D&Doom
//
// Created by <NAME> on 2016-03-23.
// Copyright © 2016 <NAME>. All rights reserved.
//
import Foundation
import UIKit
import GLKit
import OpenGLES
class RenderContext {
//Player variables
//var Ammo, Hud, Health, Weapon
init() {
}
}<file_sep>//
// SettingsTableViewController.swift
// D&Doom
//
// Created by <NAME> on 2016-02-23.
// Copyright © 2016 <NAME>. All rights reserved.
//
import Foundation
import AVFoundation
import UIKit
class SettingsTableViewController : UITableViewController
{
var settingMusicOn = false
public var themePlayer : AVAudioPlayer!;
@IBAction func settingMusicChanged(sender: AnyObject, forEvent event: UIEvent)
{
settingMusicOn = (sender as? UISwitch)?.on == true
//music code
if let path = NSBundle.mainBundle().pathForResource("Assets.xcassets/music0.dataset/1348", ofType: "mp3") {
let soundURL = NSURL(fileURLWithPath:path)
var error:NSError?
do {
themePlayer = try AVAudioPlayer(contentsOfURL: soundURL);
themePlayer.prepareToPlay()
themePlayer.numberOfLoops = -1;
themePlayer.play()
}
catch {
}
}
}
override func viewDidLoad()
{
super.viewDidLoad()
self.tableView.backgroundColor = UIColor( red: 243.0/255, green: 243.0/255, blue: 243.0/255, alpha: 1 )
}
override func didReceiveMemoryWarning()
{
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
<file_sep>// This is a .h file for the model: level
// Positions: 968
// Texels: 4
// Normals: 664
// Faces: 1922
// Vertices: 5766
const int levelVertices;
const float *levelPositions;
const float levelTexels[11532];
const float *levelNormals;
<file_sep>//
// Actor.swift
// D&Doom
//
// Created by <NAME> on 2016-02-20.
// Copyright © 2016 <NAME>. All rights reserved.
//
import Foundation
import UIKit
import GLKit
import OpenGLES
class Actor {
//Following idiom as stated from http://stackoverflow.com/questions/25918628/how-to-define-static-constant-in-a-class-in-swift
struct modelVars {
var vertexPositions : [Float] = [];
var vertexNormals : [Float] = [];
var vertexTexCoords : [Float] = [];
var vertexArray : [Float] = [];
var normalArray : [Float] = [];
init() {
}
};
var _modelVars = modelVars(); //data for model variables
struct ActorConstants {
static let _origin = GLKVector3Make(0, 0, 0);
//Don't need type name apparently
static let FPS = 60; //Placed here for now, rather than in GameViewController
static let _timeBeforeShot = 180;
}
var _position = GLKVector3Make(0, 0, 0);
var _velocity = GLKVector3Make(0, 0, 0);
var _acceleration = GLKVector3Make(0, 0, 0); // in number of seconds
var _radius = 10; //used for 'bounding spheres' collision detection; using an arbitrary default value
init(position: GLKVector3) {
_position = position;
}
func update() {
}
}<file_sep>// This is a .h file for the model: projectile
// Positions: 482
// Texels: 4
// Normals: 621
// Faces: 960
// Vertices: 2880
const int projectileVertices;
const float projectilePositions[8640];
const float projectileTexels[5760];
const float projectileNormals[8640];
<file_sep>//
// NewClass.swift
// D&Doom
//
// Created by <NAME> on 2016-02-24.
// Copyright © 2016 <NAME>. All rights reserved.
//
import Foundation
class NewClass
{
}
<file_sep>//
// Rail.swift
// D&Doom
//
// Created by <NAME> on 2016-02-24.
// Copyright © 2016 <NAME>. All rights reserved.
//
import Foundation
import GLKit
import OpenGLES
class Rail
{
var mVertices = [GLKVector3]()
}<file_sep>//
// MainViewController.swift
// D&Doom
//
// Created by <NAME> on 2016-02-10.
// Copyright © 2016 <NAME>. All rights reserved.
//
import UIKit
class MainViewController: UIViewController
{
override func shouldAutorotate() -> Bool
{
return true
}
@IBOutlet var mPlayButton: UIButton!
@IBOutlet var mOptionButton: UIButton!
@IBOutlet var mCharacterbutton: UIButton!
override func supportedInterfaceOrientations() -> UIInterfaceOrientationMask
{
return UIInterfaceOrientationMask.Landscape//UIInterfaceOrientationMask.AllButUpsideDown
}
override func viewDidLoad()
{
super.viewDidLoad()
let filepath = NSBundle.mainBundle().pathForResource("BGT1", ofType: "gif");
let gif = NSData(contentsOfFile: filepath!);
let BGP = UIWebView(frame: view.frame);
BGP.loadData(gif!, MIMEType: "image/gif", textEncodingName: String(), baseURL: NSURL());
BGP.userInteractionEnabled = false;
self.view.insertSubview(BGP, atIndex: 0)
let filter = UIWebView();
filter.frame = self.view.frame;
filter.backgroundColor = UIColor.blackColor()
filter.alpha = 0.05
self.view.addSubview(filter)
mPlayButton.translatesAutoresizingMaskIntoConstraints = true;
//let loginBtn = UIButton(frame: CGRectMake(40, 260, 240, 40))
mPlayButton.frame = CGRectMake(40, 650, 240, 90)
mPlayButton.layer.borderColor = UIColor.blackColor().CGColor
mPlayButton.layer.borderWidth = 2
mPlayButton.titleLabel!.font = UIFont.systemFontOfSize(45)
mPlayButton.tintColor = UIColor.blackColor()
mPlayButton.setTitle("Play", forState: UIControlState.Normal)
self.view.addSubview(mPlayButton)
mOptionButton.translatesAutoresizingMaskIntoConstraints = true;
//let mOptionButton = UIButton(frame: CGRectMake(40, 330, 240, 40))
mOptionButton.frame = CGRectMake(400, 650, 240, 90)
mOptionButton.layer.borderColor = UIColor.blackColor().CGColor
mOptionButton.layer.borderWidth = 2
mOptionButton.titleLabel!.font = UIFont.systemFontOfSize(45)
mOptionButton.tintColor = UIColor.blackColor()
mOptionButton.setTitle("Options", forState: UIControlState.Normal)
self.view.addSubview(mOptionButton)
mCharacterbutton.translatesAutoresizingMaskIntoConstraints = true;
//let CharacterBtn = UIButton(frame: CGRectMake(40, 400, 240, 40))
mCharacterbutton.frame = CGRectMake(750, 650, 240, 90)
mCharacterbutton.layer.borderColor = UIColor.blackColor().CGColor
mCharacterbutton.layer.borderWidth = 2
mCharacterbutton.titleLabel!.font = UIFont.systemFontOfSize(45)
mCharacterbutton.tintColor = UIColor.blackColor()
mCharacterbutton.setTitle("Character", forState: UIControlState.Normal)
self.view.addSubview(mCharacterbutton)
}
override func didReceiveMemoryWarning()
{
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
func update()
{
}
override func viewDidAppear( animated: Bool )
{
let appDelegate = UIApplication.sharedApplication().delegate as! AppDelegate
appDelegate.oriMask = supportedInterfaceOrientations()
NSNotificationCenter.defaultCenter().addObserver(
self,
selector: "rotated",
name: UIDeviceOrientationDidChangeNotification,
object: nil )
}
override func viewDidDisappear( animated: Bool )
{
let appDelegate = UIApplication.sharedApplication().delegate as! AppDelegate
appDelegate.oriMask = UIInterfaceOrientationMask.All
NSNotificationCenter.defaultCenter().removeObserver( self )
}
func rotated()
{
return
if UIDeviceOrientationIsLandscape( UIDevice.currentDevice().orientation )
{
let appDelegate = UIApplication.sharedApplication().delegate as! AppDelegate
appDelegate.oriMask = UIInterfaceOrientationMask.All
let vc = self.presentingViewController!.storyboard!.instantiateViewControllerWithIdentifier( "GameViewController" ) as! GameViewController
self.presentViewController( vc, animated: true, completion: nil )
}
}
}
<file_sep>//
// Enemy.swift
// D&Doom
//
// Created by <NAME> on 2016-03-22.
// Copyright © 2016 <NAME>. All rights reserved.
//
import Foundation
import UIKit
import GLKit
import OpenGLES
class Enemy : Actor {
//AI: Shoot every 3 seconds.
var _currTimeBeforeShot = ActorConstants._timeBeforeShot;
var _shotVelocity = 10; //In world coordinates per second
var _health = 50;
init(health: Int) {
super.init(position: ActorConstants._origin);
_health = health;
//timer ticks once every FPS frames (curr. 60 as of this writing)
//_timer = NSTimer.scheduledTimerWithTimeInterval(1.0/Double(ActorConstants.FPS), target: self, selector: "update", userInfo: nil, repeats: true);
//Don't need timer; will just use update()
}
override func update() {
//Shoot every _timeBeforeShot seconds.
if(_currTimeBeforeShot <= 0) {
//shoot projectile towards player, will need to home in as well
var vecDir = GLKVector3Subtract(GameViewController.position, self._position);
vecDir = GLKVector3Normalize(vecDir); //unit vector used to get direction of vector
let projVelocity = GLKVector3MultiplyScalar(vecDir, 10)
var mVar = modelVars();
let projectile = Projectile(position: _position, velocity: projVelocity, mVars:mVar); //constantly updating direction
//pass modelVars into it
if ((GameViewController.ActorLists[0] as? [Projectile]) != nil) { //creates a copy of the array, due to swift - http://stackoverflow.com/questions/27812433/swift-how-do-i-make-a-exact-duplicate-copy-of-an-array
//GameViewController.ActorLists[0].removeAll();
GameViewController.ActorLists[0].append(projectile); //adds to its own constructor
}
//let _vecOrigin = GLKMathUnproject(Actor.ActorConstants._origin, modelView, projection, viewport, nil);
_currTimeBeforeShot = ActorConstants._timeBeforeShot;
}
_currTimeBeforeShot--;
}
}<file_sep>// This is a .h file for the model: level0
// Positions: 20
// Texels: 44
// Normals: 9
// Faces: 22
// Vertices: 66
const int level0Vertices;
const float *level0Positions;
const float level0Texels[132];
const float *level0Normals;
<file_sep>//
// GameViewController.swift
// D&Doom
//
// Created by <NAME> on 2016-02-10.
// Copyright © 2016 <NAME>. All rights reserved.
//
import UIKit
import GLKit
import OpenGLES
import SpriteKit
import AVFoundation
func BUFFER_OFFSET( i: Int ) -> UnsafePointer< Void >
{
let p: UnsafePointer< Void > = nil
return p.advancedBy( i )
}
let UNIFORM_MODELVIEWPROJECTION_MATRIX = 0
let UNIFORM_NORMAL_MATRIX = 1
var uniforms = [GLint]( count: 2, repeatedValue: 0 )
class GameViewController: GLKViewController
{
@IBOutlet var mHud: UIView!
@IBOutlet var mAmmoLabel: UILabel!
@IBOutlet var mHealthLabel: UILabel!
@IBOutlet var mWeaponLabel: UILabel!
//For the purposes of this project, statics are a very efficient way
//with little risk of unexpected modifications.
static var mAmmo = 107;
static var mHealth = 100;
static var mWeapon = "N/A";
//Decided against a PlayerData object as well as the construct of a "RenderContext" or passing each variable to the respective classes.
//Player data
/*var Ammo : UILabel! {
get { return GameViewController.mAmmo; }
set { GameViewController.mAmmo = newValue;
mAmmoLabel = newValue;}
};*/
//var PlayerData = PlayerData();
override func shouldAutorotate() -> Bool
{
return true
}
override func supportedInterfaceOrientations() -> UIInterfaceOrientationMask
{
return UIInterfaceOrientationMask.Landscape
}
var program: GLuint = 0
//Camera stuff
var modelViewProjectionMatrix:GLKMatrix4 = GLKMatrix4Identity
var normalMatrix: GLKMatrix3 = GLKMatrix3Identity
var modelViewMatrix: GLKMatrix4 = GLKMatrix4Identity
//Accessible static var - position
static var position: GLKVector3 = GLKVector3Make(0, 0.5, 5)
var direction: GLKVector3 = GLKVector3Make(0,0,0)
var up: GLKVector3 = GLKVector3Make(0, 1, 0)
var currProjectileCoord: UILabel!;
var horizontalMovement: GLKVector3 = GLKVector3Make(0, 0, 0)
var _baseHorizontalAngle : Float = 0
var _baseVerticalAngle : Float = 0
var currhorizontalAngle: Float = 0
var currverticalAngle: Float = 0
var rotationSpeed: Float = 0.005
var vertexArray: GLuint = 0
var vertexBuffer: GLuint = 0
var context: EAGLContext? = nil
var effect: GLKBaseEffect? = nil
var _myBezier = UIBezierPath();
let bezierDuration = Float(1); //duration of bezier on screen (in seconds)
//to track swipe running or not
var _currBezierDuration = -0.00001; //hard-coded time below 0
var _currSwipeDrawn = false;
//Timer stuff (especially for enemy AI)
//var _timer : NSTimer
// @IBAction func cameraRotation(sender: UIPanGestureRecognizer) {
//
// let point: CGPoint = sender.translationInView(self.view)
//
// horizontalAngle -= Float(point.x) * rotationSpeed
//
// verticalAngle += Float(point.y) * rotationSpeed
//
// print(horizontalAngle, "h")
// print(verticalAngle, "v")
//
// sender.setTranslation(CGPointMake(0, 0), inView: self.view)
// }
@IBAction func MainButton(sender: UIButton) {
themePlayer.pause();
}
@IBAction func MoveCamera(sender: UIButton) {
GameViewController.position = GLKVector3Subtract(GameViewController.position, direction)
}
deinit
{
self.tearDownGL()
if EAGLContext.currentContext() === self.context
{
EAGLContext.setCurrentContext( nil )
}
}
// Pre-coded actors
var _PlayerShot = Actor(position: Actor.ActorConstants._origin);
//For creating a projectile.
//Tap input handling depends on variables set in update, so making this as a struct instead,
//in which update will handle the projectile creation when toCreate is set to true
//(where mouseX and mouseY are also set then)
var toCreateProjectile = false;
var mouseX : CGFloat = 0.0, mouseY : CGFloat = 0.0;
var _currProjectile = Projectile(); //a null projectile
// Hashtable storing all Lists of Actor types in the game, organized by type.
// Made static in order to be accessible by separate classes.
static var ActorLists : [Array<Actor>] = [];
static var ProjectileList : [Projectile] = [];
//For swipe action.
var _maxRadius : CGFloat = 0;
//var _maxTranslationY : CGFloat = 0;
var _prevTranslationX : CGFloat = 0; //for drawing lines
var _prevTranslationY : CGFloat = 0;
var _translationPoints : [CGPoint] = [];
var _noSwipe = false;
var _swipeHit = false;
var screenSize : CGRect = UIScreen.mainScreen().bounds;
var imageSize = CGSize(width: 200, height: 200); //arbitrary initialization
var _imageView = UIImageView();
//sound setup
// Grab the path, make sure to add it to your project!
let filePath = "footsteps_gravel";
var sound : NSURL = NSBundle.mainBundle().URLForResource("footsteps_gravel", withExtension: "wav")!;
//var audioPlayer = AVAudioPlayer()
var mySound: SystemSoundID = 0;
public var themePlayer : AVAudioPlayer!;
var soundPlayer : AVAudioPlayer!;
var soundPlayer2 : AVAudioPlayer!;
//Make an arraylist keeping track of each audio played, and remove each AVPAudioPlayer from the arraylist as each of them has completed its track, is the plan - though, still have to figure out how to set delegate and such, as to-do.
//
var AVAudioPlayers : [AVAudioPlayer] = [];
//For other iOS stuff.
typealias NSPoint = CGPoint;
typealias NSUInteger = UInt;
var _lastDate = [NSDate?](count: 64, repeatedValue: nil);
//For drawing Bezier:
//From http://stackoverflow.com/questions/10458596/bezier-curve-algorithm-in-objective-c
/* //not used
func drawBezierFrom(from:NSPoint, to:NSPoint, a:NSPoint, b:NSPoint, color:NSUInteger)
{
var qx : Float; var qy : Float;
var q1 : Float;
var q2 : Float;
var q3 : Float;
var q4 : Float;
var plotx : Float;
var ploty : Float;
var t : Float = 0.0;
while (t <= 1)
{
//Split because 'statement was too complex to be solved in a reasonable time' comes up, where it doesn't look nearly like that
q1 = Float(t*t*t*(-1) + t*t*3
+ t*(-3) + 1);
q2 = Float(t*t*t*3 + t*t*(-6)
+ t*3);
q3 = Float(t*t*t*(-3)
+ t*t*3);
q4 = Float(t*t*t);
qx = Float(q1*from.x
+ q2*a.x
+ q3*to.x
+ q4*b.x);
qy = Float(q1*from.y
+ q2*a.y
+ q3*to.y
+ q4*b.y);
plotx = round(qx);
ploty = round(qy);
self.drawPixelColor(color:color, atX:plotx, y:ploty);
t = t + 0.003;
}
}*/
//Time since the last iteration of calling this method. Original intention is for running the code, and tracking time independently of frame rate.
//Each place in code calling this is to use a different Int for 'closest-to-accurate' results (though it would not include the time after retrieving the time and updating the time, so the value would be less, or not include the time spent in retrieving the NSDate before retrieving timeDiff, ie. timeDiff would not include such time).
func timeSinceLastIter(timePointIndex: Int) -> Double {
let newTime = NSDate();
var timeDiff : Double = 0; //this would be the first time; there would be no time before this one occurred.
if(timePointIndex < _lastDate.count) {
if(_lastDate[timePointIndex] != nil) { //if an NSDate exists, then do the following
timeDiff = newTime.timeIntervalSinceDate(_lastDate[timePointIndex]!) //provided misleading error info - where I declared timeDiff resolved an error of 'not matching array type' apparently
}
}
//if let timeDiff = newTime.timeIntervalSinceDate(_lastDate[timePointIndex]);
//append array elements such that this array would be long enough to store the element at timePointIndex
if(!(timePointIndex < _lastDate.count)) {
_lastDate = _lastDate + [NSDate?](count: timePointIndex - (_lastDate.count - 1), repeatedValue: nil);
}
_lastDate[timePointIndex] = newTime;
return timeDiff;
}
//For drawing lines - from http://stackoverflow.com/questions/25229916/how-to-procedurally-draw-rectangle-lines-in-swift-using-cgcontext
func drawSwipeLine(size: CGSize) -> UIImage {
// Setup our context
let bounds = CGRect(origin: CGPoint.zero, size: size)
let opaque = false
let scale: CGFloat = 0
UIGraphicsBeginImageContextWithOptions(size, opaque, scale)
let context = UIGraphicsGetCurrentContext()
// Setup complete, do drawing here
CGContextSetStrokeColorWithColor(context, UIColor.blueColor().CGColor)
CGContextSetLineWidth(context, 4.0)
CGContextStrokeRect(context, bounds)
CGContextBeginPath(context)
let timesinceLast = timeSinceLastIter(0);
if(!_noSwipe) { //condition to erase line if swipe ended
//Draw bezier
//Maybe a cubic bezier curve?
_myBezier = UIBezierPath()
let myMicroBezier = UIBezierPath();
//Set control points c0, c1, c2, and c3 for the path myBezierPath()
var c0, c1, c2, c3 : CGPoint;
let bezierInterval = 3; //have to make sure this is divisible by 3.
//myBezier.moveToPoint(CGPoint(x: 0,y: 0));
if(!(_translationPoints.count < 1)) {
//initialization
//c0 = _translationPoints[0]; //adding this because xcode is stupid
c1 = _translationPoints[0];
c2 = _translationPoints[0];
c3 = _translationPoints[0]; //set origin point
//var currCurvePos = 1;
//draw each line, as evident from _translationPoints
for(var i=1; i < _translationPoints.count; i++) {
//CGContextMoveToPoint(context, _translationPoints[i-1].x, _translationPoints[i-1].y);
//build bezier curve
if(i%(bezierInterval/3) == 0) { //every point, add a new control point to bezier curve
//shift all of the control points by one
c0 = c1;
c1 = c2;
c2 = c3;
c3 = _translationPoints[i];
//draw the c0,c1,c2,c3 bezier curve every 3 additional control points.
if(i%(bezierInterval) == 0) { //becomes 0 ... making sometimes a straight line ... maybe the 'last line' being different in how Bezier might handle it? Oh, it's because of the closePath, and that apparently applying to addCurveToPoint ...
_myBezier.moveToPoint(c0);
_myBezier.addCurveToPoint(c3, controlPoint1: c1, controlPoint2: c2);
}
}
//get values greater than those truncated from dividing by bezierInterval, ie. values greater than the highest value quantized by bezierInterval, and draw normally according to that
let highestQuantizedVal = (_translationPoints.count / bezierInterval) * bezierInterval;
if(i > highestQuantizedVal) {
myMicroBezier.moveToPoint(_translationPoints[i-1]);
myMicroBezier.addLineToPoint(_translationPoints[i]);
}
//CGContextAddLineToPoint(context, _translationPoints[i].x, _translationPoints[i].y);
}
//draw bezier curve from those control points
_myBezier.lineWidth = 5;
//Maybe error occurs when trying to access c0 when c0 would no longer exist, ie. be out of scope?
//myBezier.addClip();
//myBezier.closePath() //may be the cause
UIColor.redColor().setStroke();
//myMicroBezier.lineWidth = 5;
//UIColor.greenColor().setStroke();
//myMicroBezier.stroke();
}
}
//Fading swipe effect
if(_currBezierDuration >= 0 && _currSwipeDrawn) {
_currBezierDuration -= timesinceLast; //reserving 0 for this
//fade only halfway through the swipe
let alpha = min(1, Float(_currBezierDuration)/Float(bezierDuration * 0.66));
UIColor(red: 1,green: 0, blue: 0, alpha:CGFloat(alpha)).setStroke();
_myBezier.stroke();
if(_currBezierDuration <= 0) {
_translationPoints.removeAll();
_currSwipeDrawn = false;
}
}
//draw min to max - so, diagonally
// CGContextMoveToPoint(context, CGRectGetMaxX(bounds), CGRectGetMinY(bounds))
// CGContextAddLineToPoint(context, CGRectGetMinX(bounds), CGRectGetMaxY(bounds))
//CGContextStrokePath(context)
// Drawing complete, retrieve the finished image and cleanup
let image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
return image
}
//animation for background color turning brown (due to earthquake)
var animationProgress : Float = 0.0; //from 0 to 1
func ThemeSound() {
if let path = NSBundle.mainBundle().pathForResource("footsteps_gravel", ofType: "wav") {
let soundURL = NSURL(fileURLWithPath:path)
var error:NSError?
do {
themePlayer = try AVAudioPlayer(contentsOfURL: soundURL);
themePlayer.prepareToPlay()
themePlayer.numberOfLoops = -1;
themePlayer.play()
}
catch {
}
}
}
override func viewDidLoad()
{
super.viewDidLoad()
self.context = EAGLContext( API: .OpenGLES2 )
if self.context == nil
{
print("Failed to create ES context")
}
GameViewController.ActorLists = [GameViewController.ProjectileList]; //Can assign an array of a subclass to an array of its superclass, apparently
let view = self.view as! GLKView
view.context = self.context!
view.drawableDepthFormat = .Format24
//mAmmoLabel.text = GameViewController.mAmmo;
mHealthLabel.text = "77%"
mWeaponLabel.text = "N/A"
animationProgress = 1;
//handle tap
let tapGesture = UITapGestureRecognizer(target: self, action: Selector("handleTapGesture:"));
tapGesture.numberOfTapsRequired = 1;
view.addGestureRecognizer(tapGesture);
//handle pan
let panGesture = UIPanGestureRecognizer(target: self, action: Selector("handlePanGesture:"));
view.addGestureRecognizer(panGesture);
//play looping sound
//From http://stackoverflow.com/questions/30873056/ios-swift-2-0-avaudioplayer-is-not-playing-any-sound
ThemeSound()
currProjectileCoord = UILabel(frame: CGRectMake(screenSize.height/2 - 100, screenSize.width - 27, 500, 21))
//currProjectileCoord.center = CGPointMake(160, 284)
//currProjectileCoord.textAlignment = NSTextAlignment.Center
currProjectileCoord.text = "Projectile Coords"
self.view.addSubview(currProjectileCoord)
self.setupGL()
}
/*func replaySound() {
AudioServicesPlaySystemSound(mySound);
}*/
//func playSound(inout soundPlayer? : AVAudioPlayer) {
//}
func cameraMovement()
{
var horizontalAngle = _baseHorizontalAngle + currhorizontalAngle;
var verticalAngle = _baseVerticalAngle + currverticalAngle;
//for animationProgress of shake
if(animationProgress > 1) {
animationProgress = 1;
}
if(animationProgress < 1) {
animationProgress += Float(1.0)/Float(45.0);
var shakeMag : Float;
if(animationProgress < 0.70) {
shakeMag = 0.9 * 0.4;
}
else {
shakeMag = (0.9 - (animationProgress - 0.7) * 0.9 / 0.3) * 0.4; //after reaching 0.7 progress (when sound starts to dwindle), linearly decrease max magnitude to 0
}
//modelViewMatrix = GLKMatrix4Translate(modelViewMatrix, Float(arc4random())*shakeMag, Float(arc4random())*shakeMag, 0);
//GLKVector3Make(position.x + Float(arc4random())*shakeMag, position.y + Float(arc4random())*shakeMag, position.z + Float(arc4random())*shakeMag);
horizontalAngle += (Float(arc4random()) / Float(UINT32_MAX)) * Float(shakeMag);
verticalAngle += (Float(arc4random()) / Float(UINT32_MAX)) * Float(shakeMag);
}
direction = GLKVector3Make(cosf(verticalAngle) * sinf(horizontalAngle),
sinf(verticalAngle),
cosf(verticalAngle) * cosf(horizontalAngle));
horizontalMovement = GLKVector3Make(sinf(horizontalAngle - Float(M_PI_2)), 0, cosf(horizontalAngle - Float(M_PI_2)));
//print("horizontalAngle: \(horizontalAngle); verticalAngle: \(verticalAngle)");
}
//Tap input event handler
//For shooting a projectile
func handleTapGesture(recognizer : UITapGestureRecognizer) {
let location : CGPoint = recognizer.locationInView(self.view);
//Act set toCreateProjectile to signal that this is to be done.
toCreateProjectile = true;
let screenSize : CGRect = UIScreen.mainScreen().bounds;
//mouseX and mouseY, where this is converted from screen to cartesian coords
mouseX = location.x - screenSize.width / 2;
mouseY = -location.y + screenSize.height / 2;
//var newProjectile = Projectile(location.x, location.y, 0, 30);
//Need model, view, and projection for the projectile.
//Play sound
if let path = NSBundle.mainBundle().pathForResource("'flyby'", ofType: "wav") {
let soundURL = NSURL(fileURLWithPath:path)
var error:NSError?
do {
soundPlayer = try AVAudioPlayer(contentsOfURL: soundURL);
soundPlayer.prepareToPlay()
//No loops
soundPlayer.play()
}
catch {
}
}
}
func handlePanGesture(recognizer : UIPanGestureRecognizer) {
let translation = recognizer.translationInView(self.view); //reusing, if the method could only be called once per recognize ... oh, was due to it being point.
let location = recognizer.locationInView(self.view);
//Actually, just get furthest radius from the origin.
let radiusVec = GLKVector2Make(Float(translation.x), Float(translation.y));
let radLength = CGFloat(GLKVector2Length(radiusVec))
if(recognizer.state == UIGestureRecognizerState.Began) {
_maxRadius = 0;
//_maxTranslationY = 0;
_noSwipe = false;
_translationPoints.removeAll();
}
if(recognizer.state == UIGestureRecognizerState.Ended) {
_noSwipe = false;
if(radLength >= 80) { //valid swipe
var swipeSound : String;
var swipeSoundExt : String = "mp3";
if(_swipeHit) {
swipeSound = "sword-clash1"
}
else {
swipeSound = "swipe_whiff";
}
if let path = NSBundle.mainBundle().pathForResource(swipeSound, ofType: swipeSoundExt) {
let soundURL = NSURL(fileURLWithPath:path)
var error:NSError?
do {
soundPlayer2 = try AVAudioPlayer(contentsOfURL: soundURL);
soundPlayer2.prepareToPlay()
soundPlayer2.play()
}
catch {
}
}
_currBezierDuration = Double(bezierDuration);
_currSwipeDrawn = true;
}
}
if(radLength > _maxRadius) {
_maxRadius = radLength;
}
//cancel gesture if moving backwards from the furthest radius from the origin (as opposed to total translation) by 6px.
//So yes, you can zigzag a lot if you wanted to.
if(radLength < _maxRadius - 6) {
//_noSwipe = true;
}
//draw line
//ie. create the _translationPoints
if(!_noSwipe) {
_translationPoints.append(CGPoint(x: location.x, y: location.y));
}
//print("radLength: \(radLength); _maxRadius: \(_maxRadius)");
//let point: CGPoint = recognizer.translationInView(self.view)
if(recognizer.state == UIGestureRecognizerState.Began) {
//_prevHorizontalAngle
_baseHorizontalAngle += currhorizontalAngle; //had missed the + increment over the previous ...
_baseVerticalAngle += currverticalAngle;
//currhorizontalAngle = 0;
//currverticalAngle = 0;
}
currhorizontalAngle = -Float(translation.x) * rotationSpeed;
currverticalAngle = Float(translation.y) * rotationSpeed;
//print(horizontalAngle, "h")
//print(verticalAngle, "v")
//recognizer.setTranslation(CGPointMake(0, 0), inView: self.view) //keeps setting it to 0; should just be the value?
}
override func canBecomeFirstResponder() -> Bool {
return true;
}
//Jacob: Shake input handler
override func motionEnded(motion: UIEventSubtype, withEvent event: UIEvent?) {
if motion == .MotionShake { //just having earthquake for now
//shake method here
//Cast spell
animationProgress = 0; //begins the animation of earthquake
if let path = NSBundle.mainBundle().pathForResource("magic-quake2", ofType: "mp3") {
let soundURL = NSURL(fileURLWithPath:path)
var error:NSError?
do {
soundPlayer = try AVAudioPlayer(contentsOfURL: soundURL);
soundPlayer.prepareToPlay()
//No loops
soundPlayer.play()
}
catch {
}
}
//Right now, it simply shakes the camera, but maybe shaking the world instead could be considered?
}
}
override func didReceiveMemoryWarning()
{
super.didReceiveMemoryWarning()
if self.isViewLoaded() && (self.view.window != nil)
{
self.view = nil
self.tearDownGL()
if EAGLContext.currentContext() === self.context
{
EAGLContext.setCurrentContext( nil )
}
self.context = nil
}
}
override func viewDidLayoutSubviews()
{
if !(mHud.subviews.first is UIVisualEffectView)
{
let blurEffect = UIBlurEffect( style: .Light )
let blurEffectView = UIVisualEffectView( effect: blurEffect )
let vibeEffectView = UIVisualEffectView( effect: UIVibrancyEffect( forBlurEffect: blurEffect ) )
blurEffectView.frame = mHud.bounds
vibeEffectView.frame = mHud.bounds
blurEffectView.addSubview( vibeEffectView )
mHud.insertSubview( blurEffectView, atIndex: 0 )
}
//Update image for lines
screenSize = UIScreen.mainScreen().bounds;
imageSize = CGSize(width: screenSize.width, height: screenSize.height);
_imageView = UIImageView(frame: CGRect(origin: CGPoint(x: 0, y: 0), size: imageSize))
self.view.addSubview(_imageView)
let image = drawSwipeLine(imageSize)
//_imageView.
_imageView.image = image
}
override func viewDidAppear( animated: Bool )
{
let appDelegate = UIApplication.sharedApplication().delegate as! AppDelegate
appDelegate.oriMask = supportedInterfaceOrientations()
NSNotificationCenter.defaultCenter().addObserver(
self,
selector: "rotated",
name: UIDeviceOrientationDidChangeNotification,
object: nil )
}
override func viewDidDisappear( animated: Bool )
{
let appDelegate = UIApplication.sharedApplication().delegate as! AppDelegate
appDelegate.oriMask = UIInterfaceOrientationMask.All
NSNotificationCenter.defaultCenter().removeObserver( self )
}
func rotated()
{
/*if UIDeviceOrientationIsPortrait( UIDevice.currentDevice().orientation )
{
let appDelegate = UIApplication.sharedApplication().delegate as! AppDelegate
appDelegate.oriMask = UIInterfaceOrientationMask.All
let vc = self.presentingViewController!.storyboard!.instantiateViewControllerWithIdentifier( "MainViewController" ) as! MainViewController
self.presentViewController( vc, animated: true, completion: nil )
}*/
}
// BOILERPLATE
func setupGL()
{
EAGLContext.setCurrentContext( self.context )
self.loadShaders()
self.effect = GLKBaseEffect()
self.effect!.light0.enabled = GLboolean( GL_TRUE )
self.effect!.light0.diffuseColor = GLKVector4Make( 1.0, 0.4, 0.4, 1.0 )
glEnable( GLenum( GL_DEPTH_TEST ) )
glGenVertexArraysOES( 1, &vertexArray )
glBindVertexArrayOES( vertexArray )
glGenBuffers( 1, &vertexBuffer )
glBindBuffer( GLenum( GL_ARRAY_BUFFER ), vertexBuffer )
//glBufferData( GLenum( GL_ARRAY_BUFFER ), GLsizeiptr( sizeof( GLfloat ) * gCubeVertexData.count ), &gCubeVertexData, GLenum( GL_STATIC_DRAW ) )
glEnableVertexAttribArray( GLuint( GLKVertexAttrib.Position.rawValue ) )
glVertexAttribPointer( GLuint( GLKVertexAttrib.Position.rawValue ), 3, GLenum( GL_FLOAT ), GLboolean( GL_FALSE ), 0, levelPositions)
glEnableVertexAttribArray( GLuint( GLKVertexAttrib.Normal.rawValue ) )
glVertexAttribPointer( GLuint( GLKVertexAttrib.Normal.rawValue ), 3, GLenum( GL_FLOAT ), GLboolean( GL_FALSE ), 24, levelNormals )
glBindVertexArrayOES( 0 )
}
func tearDownGL()
{
EAGLContext.setCurrentContext( self.context )
glDeleteBuffers( 1, &vertexBuffer )
glDeleteVertexArraysOES( 1, &vertexArray )
self.effect = nil
if program != 0
{
glDeleteProgram( program )
program = 0
}
}
// MARK: - GLKView and GLKViewController delegate methods
func update()
{
let aspect = fabsf( Float( self.view.bounds.size.width / self.view.bounds.size.height ) )
let projectionMatrix = GLKMatrix4MakePerspective( GLKMathDegreesToRadians( 65.0 ), aspect, 0.1, 100.0 )
//get inverse of seconds per update call
//(roughly)
//let timeSinceLastDate = NSDate().timeIntervalSinceDate(_currDate); // aka 'seconds / update'
let UPS = 1 / timeSinceLastIter(1);
self.effect?.transform.projectionMatrix = projectionMatrix
self.cameraMovement();
//var newPos : GLKVector3;
//This were used to orient the matrix.
modelViewMatrix = GLKMatrix4MakeLookAt(GameViewController.position.x, GameViewController.position.y, GameViewController.position.z,
GLKVector3Subtract(GameViewController.position, direction).x,
GLKVector3Subtract(GameViewController.position, direction).y,
GLKVector3Subtract(GameViewController.position, direction).z,
up.x, up.y, up.z);
//self.effect?. = UIColor.brownColor().colorWithAlphaComponent(CGFloat(1 - animationProgress)); //set background color
modelViewProjectionMatrix = GLKMatrix4Multiply( projectionMatrix, modelViewMatrix )
//modelViewMatrix = GLKMatrix4Multiply( baseModelViewMatrix, modelViewMatrix )
//modelViewProjectionMatrix = GLKMatrix4Multiply( projectionMatrix, modelViewMatrix )
self.effect?.transform.modelviewMatrix = modelViewMatrix;
//Still have to rotate the projectile velocity and vectors to make them facing direction of the camera; currently the projectile's projectile as if the camera were from 0,0,0 to facing towards the center of the screen, unless Unproject takes the view (which would involve the camera angle) into account.
//Test model, view, and projection for projectile
if(toCreateProjectile) {
let StartProjectile = Projectile.ActorConstants._origin;
let screenSize : CGRect = UIScreen.mainScreen().bounds;
let viewport = UnsafeMutablePointer<Int32>([Int32(0), Int32(screenSize.height - 100), Int32(screenSize.width), Int32(screenSize.height)]);
//TODO: Set modelVars for projectileModel
let projectileModel = Actor.modelVars();
let projectile = Projectile(screenX: mouseX, screenY: mouseY, farplaneZ: Int(100), speed: 5, modelView: modelViewMatrix, projection: projectionMatrix, viewport: viewport, mVars: projectileModel);
//Casting ActorLists[0] as [Projectile], getting the reference of that
if ((GameViewController.ActorLists[0] as? [Projectile]) != nil) { //creates a copy of the array, due to swift - http://stackoverflow.com/questions/27812433/swift-how-do-i-make-a-exact-duplicate-copy-of-an-array
GameViewController.ActorLists[0].removeAll();
GameViewController.ActorLists[0].append(projectile); //adds to its own constructor
}
toCreateProjectile = false;
//projectile.printVector("a", vec: projectile._velocity);
_currProjectile = projectile;
}
//GameViewController.ActorLists[0].append(projectile);
//GameViewController.ProjectileList.append(projectile);
currProjectileCoord.text = "Projectile Pos'n: (\(_currProjectile._position.x),\(_currProjectile._position.y),\(_currProjectile._position.z))";
//update HUD values. Other than reassignment each frame, would have to maybe pass by reference, but that doesn't look possible.
mAmmoLabel.text = String(GameViewController.mAmmo);
mHealthLabel.text = String(GameViewController.mHealth) + "%";
mWeaponLabel.text = GameViewController.mWeapon;
//update line
let image = drawSwipeLine(imageSize)
_imageView.image = image
//Update all Actors in ActorLists
for ActorList in GameViewController.ActorLists {
for actor in ActorList as! [Actor] { //force downcast/unwrap with "as!"
actor.update(); //actor...
}
}
}
override func glkView( view: GLKView, drawInRect rect: CGRect )
{
glClearColor( 0.65, 0.65, 0.65, 1.0 )
glClear( GLbitfield( GL_COLOR_BUFFER_BIT ) | GLbitfield( GL_DEPTH_BUFFER_BIT ) )
glBindVertexArrayOES( vertexArray )
// Render the object with GLKit
self.effect?.prepareToDraw()
//glDrawArrays( GLenum( GL_TRIANGLES ) , 0, 36 )
// Render the object again with ES2
glUseProgram( program )
withUnsafePointer( &modelViewProjectionMatrix, {
glUniformMatrix4fv( uniforms[ UNIFORM_MODELVIEWPROJECTION_MATRIX ], 1, 0, UnsafePointer( $0 ) )
} )
withUnsafePointer( &normalMatrix, {
glUniformMatrix3fv( uniforms[ UNIFORM_NORMAL_MATRIX ], 1, 0, UnsafePointer( $0 ) )
} )
glDrawArrays( GLenum( GL_TRIANGLES ), 0, levelVertices )
}
// MARK: - OpenGL ES 2 shader compilation
func loadShaders() -> Bool
{
var vertShader: GLuint = 0
var fragShader: GLuint = 0
var vertShaderPathname: String
var fragShaderPathname: String
// Create shader program.
program = glCreateProgram()
// Create and compile vertex shader.
vertShaderPathname = NSBundle.mainBundle().pathForResource( "Shader", ofType: "vsh" )!
if self.compileShader( &vertShader, type: GLenum( GL_VERTEX_SHADER ), file: vertShaderPathname ) == false
{
print( "Failed to compile vertex shader" )
return false
}
// Create and compile fragment shader.
fragShaderPathname = NSBundle.mainBundle().pathForResource( "Shader", ofType: "fsh" )!
if !self.compileShader( &fragShader, type: GLenum( GL_FRAGMENT_SHADER ), file: fragShaderPathname )
{
print( "Failed to compile fragment shader" )
return false
}
// Attach vertex shader to program.
glAttachShader( program, vertShader )
// Attach fragment shader to program.
glAttachShader( program, fragShader )
// Bind attribute locations.
// This needs to be done prior to linking.
glBindAttribLocation( program, GLuint( GLKVertexAttrib.Position.rawValue ), "position" )
glBindAttribLocation( program, GLuint( GLKVertexAttrib.Normal.rawValue ), "normal" )
// Link program.
if !self.linkProgram( program )
{
print( "Failed to link program: \(program)" )
if vertShader != 0
{
glDeleteShader( vertShader )
vertShader = 0
}
if fragShader != 0
{
glDeleteShader( fragShader )
fragShader = 0
}
if program != 0
{
glDeleteProgram( program )
program = 0
}
return false
}
// Get uniform locations.
uniforms[ UNIFORM_MODELVIEWPROJECTION_MATRIX ] = glGetUniformLocation( program, "modelViewProjectionMatrix" )
uniforms[ UNIFORM_NORMAL_MATRIX ] = glGetUniformLocation( program, "normalMatrix" )
// Release vertex and fragment shaders.
if vertShader != 0
{
glDetachShader( program, vertShader )
glDeleteShader( vertShader )
}
if fragShader != 0
{
glDetachShader( program, fragShader )
glDeleteShader( fragShader )
}
return true
}
func compileShader( inout shader: GLuint, type: GLenum, file: String ) -> Bool
{
var status: GLint = 0
var source: UnsafePointer< Int8 >
do {
source = try NSString( contentsOfFile: file, encoding: NSUTF8StringEncoding ).UTF8String
} catch {
print( "Failed to load vertex shader" )
return false
}
var castSource = UnsafePointer< GLchar >( source )
shader = glCreateShader( type )
glShaderSource( shader, 1, &castSource, nil )
glCompileShader( shader )
//#if defined( DEBUG )
// var logLength: GLint = 0
// glGetShaderiv( shader, GLenum( GL_INFO_LOG_LENGTH ), &logLength )
// if logLength > 0
// {
// var log = UnsafeMutablePointer< GLchar >( malloc( Int( logLength ) ) )
// glGetShaderInfoLog( shader, logLength, &logLength, log )
// NSLog( "Shader compile log: \n%s", log )
// free( log )
// }
//#endif
glGetShaderiv( shader, GLenum( GL_COMPILE_STATUS ), &status )
if status == 0
{
glDeleteShader( shader )
return false
}
return true
}
func linkProgram( prog: GLuint ) -> Bool
{
var status: GLint = 0
glLinkProgram( prog )
//#if defined( DEBUG )
// var logLength: GLint = 0
// glGetShaderiv( shader, GLenum( GL_INFO_LOG_LENGTH ), &logLength )
// if logLength > 0
// {
// var log = UnsafeMutablePointer< GLchar >( malloc( Int( logLength ) ) )
// glGetShaderInfoLog( shader, logLength, &logLength, log )
// NSLog( "Shader compile log: \n%s", log )
// free( log )
// }
//#endif
glGetProgramiv( prog, GLenum( GL_LINK_STATUS ), &status )
return status != 0
}
func validateProgram( prog: GLuint ) -> Bool
{
var logLength: GLsizei = 0
var status: GLint = 0
glValidateProgram( prog )
glGetProgramiv( prog, GLenum( GL_INFO_LOG_LENGTH ), &logLength )
if logLength > 0
{
var log: [GLchar] = [GLchar]( count: Int( logLength ), repeatedValue: 0 )
glGetProgramInfoLog( prog, logLength, &logLength, &log )
print( "Program validate log: \n\(log)" )
}
glGetProgramiv( prog, GLenum( GL_VALIDATE_STATUS ), &status )
return status != 0
}
}
var gCubeVertexData: [GLfloat] = [
// Data layout for each line below is:
// positionX, positionY, positionZ, normalX, normalY, normalZ,
0.5, -0.5, -0.5, 1.0, 0.0, 0.0,
0.5, 0.5, -0.5, 1.0, 0.0, 0.0,
0.5, -0.5, 0.5, 1.0, 0.0, 0.0,
0.5, -0.5, 0.5, 1.0, 0.0, 0.0,
0.5, 0.5, -0.5, 1.0, 0.0, 0.0,
0.5, 0.5, 0.5, 1.0, 0.0, 0.0,
0.5, 0.5, -0.5, 0.0, 1.0, 0.0,
-0.5, 0.5, -0.5, 0.0, 1.0, 0.0,
0.5, 0.5, 0.5, 0.0, 1.0, 0.0,
0.5, 0.5, 0.5, 0.0, 1.0, 0.0,
-0.5, 0.5, -0.5, 0.0, 1.0, 0.0,
-0.5, 0.5, 0.5, 0.0, 1.0, 0.0,
-0.5, 0.5, -0.5, -1.0, 0.0, 0.0,
-0.5, -0.5, -0.5, -1.0, 0.0, 0.0,
-0.5, 0.5, 0.5, -1.0, 0.0, 0.0,
-0.5, 0.5, 0.5, -1.0, 0.0, 0.0,
-0.5, -0.5, -0.5, -1.0, 0.0, 0.0,
-0.5, -0.5, 0.5, -1.0, 0.0, 0.0,
-0.5, -0.5, -0.5, 0.0, -1.0, 0.0,
0.5, -0.5, -0.5, 0.0, -1.0, 0.0,
-0.5, -0.5, 0.5, 0.0, -1.0, 0.0,
-0.5, -0.5, 0.5, 0.0, -1.0, 0.0,
0.5, -0.5, -0.5, 0.0, -1.0, 0.0,
0.5, -0.5, 0.5, 0.0, -1.0, 0.0,
0.5, 0.5, 0.5, 0.0, 0.0, 1.0,
-0.5, 0.5, 0.5, 0.0, 0.0, 1.0,
0.5, -0.5, 0.5, 0.0, 0.0, 1.0,
0.5, -0.5, 0.5, 0.0, 0.0, 1.0,
-0.5, 0.5, 0.5, 0.0, 0.0, 1.0,
-0.5, -0.5, 0.5, 0.0, 0.0, 1.0,
0.5, -0.5, -0.5, 0.0, 0.0, -1.0,
-0.5, -0.5, -0.5, 0.0, 0.0, -1.0,
0.5, 0.5, -0.5, 0.0, 0.0, -1.0,
0.5, 0.5, -0.5, 0.0, 0.0, -1.0,
-0.5, -0.5, -0.5, 0.0, 0.0, -1.0,
-0.5, 0.5, -0.5, 0.0, 0.0, -1.0
]
| c70e3ad5b8347f58ffcc1e630bc6431f223f97c1 | [
"Swift",
"C"
] | 12 | Swift | theLOLflashlight/D-Doom | 3da1d01574434b538ad8a0a35afd672a39712ba4 | 5518b387937c112a5f4ed6d6faf7f7cdea3b74a9 | |
refs/heads/master | <repo_name>theerincollins/dictionary<file_sep>/lib/definition.rb
class Definition
@@definitions = []
attr_reader(:description)
define_method(:initialize) do |description|
@description = description
end
define_method(:save) do
@@definitions.push(self)
end
define_singleton_method(:clear) do
@@definitions = []
end
define_singleton_method(:all) do
@@definitions
end
end
<file_sep>/spec/dictionary_integration_spec.rb
require ('capybara/rspec')
require('./app')
Capybara.app = Sinatra::Application
set(:show_exceptions, false)
describe('add new word path', {:type => :feature}) do
it('show page for input a new word and then display that word with all other words in the dictionary') do
visit('/word/new')
fill_in('word_name', :with => 'Airplane')
click_button('+ Add')
expect(page).to have_content('Airplane')
end
end
describe('add new definition path', {:type => :feature}) do
it('show page a word that exists, a user can input a definition and it will be added to that word') do
visit('/word/new')
fill_in('definition', :with => 'wow')
click_button('+ Add')
expect(page).to have_content('wow')
end
end
<file_sep>/README.md
Dictionary
Description: Using Ruby this method will list the words in a dictionary. A user can select a word to see the definitions and add more definitions to existing words. A user can also add a new word and definition.
The method will alert the user if they enter a word twice.
Author: <NAME> Date: May 1, 2015
Link to Heroku: https://erin-dictionary.herokuapp.com/word/new
Setup Instructions: -- Download all files -- Run bundle install -- Run Ruby app.rb -- Enter localhost:4567 into address bar
The MIT License
Copyright (c) 2015 <NAME> <EMAIL>
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
<file_sep>/spec/dictionary_spec.rb
require('rspec')
require('word')
require('definition')
describe Word do
before do
Word.clear()
end
describe('.all') do
it('will return array of all words') do
test_word = Word.new("cheeseburger").save()
expect(Word.all).to(eq(test_word))
end
it('will be empty at first') do
expect(Word.all).to(eq([]))
end
end
describe('.clear') do
it('will clear out array of words') do
test_word = Word.new("cheeseburger").save()
Word.clear()
expect(Word.all).to(eq([]))
end
end
describe('#save') do
it('will save a word to an array of all words') do
test_word = Word.new("vegan")
test_word.save()
expect(Word.all).to(eq([test_word]))
end
end
describe('#id') do
it('will return id of a word') do
test_word = Word.new("Snoopy")
expect(test_word.id()).to(eq(1))
end
end
describe('#name') do
it('will return the name of a word') do
test_word = Word.new("Peanut")
expect(test_word.word_name()).to(eq("Peanut"))
end
end
describe('.find') do
it("will return a word by using that word's id") do
test_word1 = Word.new("Hard")
test_word1.save()
test_word2 = Word.new("Rock")
test_word2.save()
expect(Word.find(test_word2.id())).to(eq(test_word2))
end
end
describe('#add_definition') do
it("will add a definition to a word's array of definitions") do
test_definition1 = Definition.new("it barks a lot")
test_definition2 = Definition.new("it pees a lot")
test_word = Word.new("dog")
test_word.save()
test_word.add_definition(test_definition1)
test_word.add_definition(test_definition2)
expect(test_word.get_definitions()).to(eq([test_definition1, test_definition2]))
end
end
describe('.duplicate?') do
it('will return true if the word entered is alread in the dictionary') do
test_word1 = Word.new("dog")
test_word1.save()
test_word2 = Word.new("dog")
test_word2.save()
expect(Word.duplicate?(test_word2.word_name())).to(eq(true))
end
end
describe('.delete_duplicate') do
it('will delete the last word if it was a duplicate') do
test_word1 = Word.new("cat")
test_word1.save()
test_word2 = Word.new("dog")
test_word2.save()
test_word3 = Word.new("dog")
test_word3.save()
Word.delete_duplicate(test_word3)
expect(Word.all).to(eq([test_word1, test_word2]))
end
end
end
describe Definition do
before do
Definition.clear()
end
describe('.clear') do
it('will clear array of definitions') do
Definition.new("this and that").save()
Definition.clear
expect(Definition.all()).to(eq([]))
end
end
describe('.all') do
it('will be empty at first') do
expect(Definition.all()).to(eq([]))
end
end
describe('#save') do
it('will save a definition to an array of definitions') do
test_definition = Definition.new("those things over there")
test_definition.save()
expect(Definition.all).to(eq([test_definition]))
end
end
describe('#description') do
it('will return the description of the definition') do
test_definition = Definition.new("those things over there")
expect(test_definition.description).to(eq("those things over there"))
end
end
end
<file_sep>/lib/word.rb
class Word
@@words = []
attr_reader(:word_name, :id)
define_method(:initialize) do |word_name|
@word_name = word_name
@id = @@words.length() + 1
@word_definitions = []
end
define_singleton_method(:clear) do
@@words = []
end
define_singleton_method(:all) do
@@words
end
define_singleton_method(:find) do |id|
found_word = ''
@@words.each do |word|
if word.id() == id
found_word = word
end
end
found_word
end
define_method(:save) do
@@words.push(self)
end
define_method(:add_definition) do |definition|
@word_definitions.push(definition)
end
define_method(:get_definitions) do
@word_definitions
end
define_singleton_method(:duplicate?) do |word_name|
duplicate = nil
@@words.each do |word|
if word.word_name == word_name
duplicate = true
end
end
duplicate
end
define_singleton_method(:delete_duplicate) do |word|
if Word.duplicate?(word.word_name())
@@words.pop
end
@@words
end
end
<file_sep>/app.rb
require('./lib/word')
require('./lib/definition')
require('sinatra')
require('sinatra/reloader')
also_reload('lib/**/*.rb')
require('pry')
require('rubygems')
get('/') do
@words = Word.all
erb(:index)
end
get('/word/new') do
erb(:new_word)
end
post('/save_word') do
@new_word = Word.new(params.fetch("word_name"))
definition = Definition.new(params.fetch("definition"))
@new_word.add_definition(definition)
@definitions = @new_word.get_definitions()
@duplicate = Word.duplicate?(@new_word.word_name())
@new_word.save()
erb(:word)
end
get('/word/:id') do
@new_word = Word.find(params.fetch('id').to_i())
@definitions = @new_word.get_definitions()
erb(:word)
end
post('/add_definition') do
new_definition = Definition.new(params.fetch("definition"))
@new_word = Word.find(params.fetch("word_id").to_i())
@new_word.add_definition(new_definition)
@definitions = @new_word.get_definitions()
erb(:word)
end
get('/reset') do
Word.clear()
erb(:index)
end
| c00ab157c36ebb2eb8e54c53608686b2d734a699 | [
"Markdown",
"Ruby"
] | 6 | Ruby | theerincollins/dictionary | f98170f2356a239881ca420122a4a5933854d63b | a25f36f61cd81986cf4af498330b628f221fa3f0 | |
refs/heads/master | <file_sep>public class throwbackanexception {
public int someMethod2(int money) throws Exception {
if (money < 1)
throw new ArithmeticException("Error: amount too small");
return money;
}
public int someMethod() throws Exception {
throwbackanexception a = new throwbackanexception();
int b = a.someMethod2(1) + 20;
return b;
}
public static void main(String[] args) {
try {
throwbackanexception a = new throwbackanexception();
int t = a.someMethod();
System.out.println("" + t);
} catch (Exception e) {
System.out.println("Error:");
e.printStackTrace();
}
}
}
| ed5744a1e36eef4e68de430735db058021513aaa | [
"Java"
] | 1 | Java | trungdh/asg08 | c9e8a9852d03332b3a3434f2cb1aae110ee225c0 | ab6bd7959929bf09cea17a3167ed503c8904587b | |
refs/heads/master | <repo_name>PhoenixFate/darkhorse297-jpa<file_sep>/spring-data-jpa/src/test/java/com/phoenix/test/CustomerObjectNavigationTest.java
package com.phoenix.test;
import com.phoenix.dao.CustomerDao;
import com.phoenix.dao.LinkManDao;
import com.phoenix.domain.Customer;
import com.phoenix.domain.LinkMan;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.annotation.Rollback;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import org.springframework.transaction.annotation.Transactional;
import java.util.Set;
/**
* important
* 对象导航查询
*/
@RunWith(SpringJUnit4ClassRunner.class) //声明spring提供的单元测试环境
@ContextConfiguration(locations = "classpath:applicationContext.xml") //指定spring容器的配置信息
public class CustomerObjectNavigationTest {
@Autowired
private CustomerDao customerDao;
@Autowired
private LinkManDao linkManDao;
/**
* 测试对象导航(查询一个对象的时候,通过此对象查询所有的关联对象)
*/
@Test
@Transactional
@Rollback(value = false)
public void testObjectNavigation(){
//getOne() 延迟加载
Customer customer = customerDao.getOne(5L);
//对象导航查询
Set<LinkMan> linkMans = customer.getLinkMans();
for(LinkMan linkMan:linkMans){
System.out.println(linkMan);
}
}
/**
* 测试对象导航(查询一个对象的时候,通过此对象查询所有的关联对象)
*
* 对象导航查询
* 默认使用的是延迟加载的形式查询的
* 调用get方法并不会立即发送查询,而是在使用关联查询对象的时候才会延迟查询
* 可修改配置,将延迟加载改为立即加载
* fetch,需要配置到多表映射的关系的注解上
*/
@Test
@Transactional
@Rollback(value = false)
public void testObjectNavigation2(){
//findOne() 立即加载
Customer customer = customerDao.findOne(5L);
//对象导航查询
Set<LinkMan> linkMans = customer.getLinkMans();
for(LinkMan linkMan:linkMans){
System.out.println(linkMan);
}
}
/**
* 从多的一方查询一的一方,默认是立即加载
* 从联系人对象导航查询他所属的客户
*/
@Test
@Transactional
@Rollback(value = false)
public void testObjectNavigation3(){
LinkMan linkMan = linkManDao.findOne(1L);
Customer customer = linkMan.getCustomer();
System.out.println(customer);
}
}
<file_sep>/spring-data-jpa/src/test/java/com/phoenix/test/CustomerSqlTest.java
package com.phoenix.test;
import com.phoenix.dao.CustomerDao;
import com.phoenix.domain.Customer;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.annotation.Rollback;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import org.springframework.transaction.annotation.Transactional;
import java.util.List;
@RunWith(SpringJUnit4ClassRunner.class) //声明spring提供的单元测试环境
@ContextConfiguration(locations = "classpath:applicationContext.xml") //指定spring容器的配置信息
public class CustomerSqlTest {
@Autowired
private CustomerDao customerDao;
@Test
public void testFindAllBySql(){
List<Customer> customerList = customerDao.findAllBySql();
for(Customer customer:customerList){
System.out.println(customer);
}
}
@Test
public void testFindByNameBySql(){
List<Customer> customerList=customerDao.findByNameBySql("%tomcat%");
for(Customer customer:customerList){
System.out.println(customer);
}
}
}
<file_sep>/spring-data-jpa/src/test/java/com/phoenix/test/CustomerTest.java
package com.phoenix.test;
import com.phoenix.dao.CustomerDao;
import com.phoenix.domain.Customer;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import org.springframework.transaction.annotation.Transactional;
import java.util.List;
@RunWith(SpringJUnit4ClassRunner.class) //声明spring提供的单元测试环境
@ContextConfiguration(locations = "classpath:applicationContext.xml") //指定spring容器的配置信息
public class CustomerTest {
@Autowired
private CustomerDao customerDao;
@Test
public void testFindAll(){
List<Customer> customerList = customerDao.findAll();
for(Customer customer:customerList){
System.out.println(customer);
}
}
@Test
public void testFindOne(){
Customer one = customerDao.findOne(2l);
System.out.println(one);
}
/**
* save 保存或者更新
* 根据传递的对象释放存在主键id
* 如果没有id主键属性:保存
* 存在id主键属性,则根据id查询数据,更新数据
*/
@Test
public void testSave(){
Customer customer=new Customer();
customer.setCustName("spring data jps");
customer.setCustAddress("spring address");
customer.setCustIndustry("spring industry");
customerDao.save(customer);
}
@Test
public void testUpdate(){
Customer customer = customerDao.findOne(7L);
customer.setCustLevel("new level");
customerDao.save(customer);
}
@Test
public void testDelete(){
customerDao.delete(7L);
}
/**
* 统计查询,查询总数
*/
@Test
public void testCount(){
long count = customerDao.count();
System.out.println(count);
}
/**
* 判断id为4的客户是否存在
*/
@Test
public void testExists(){
boolean exists = customerDao.exists(4L);
System.out.println("id 为4的客户是否存在:"+exists);
}
/**
* getOne()
* 需要在方法名上面+ @transactional 保证正常运行
*
* findOne():
* entityManager.find() 立即加载
*
* getOne():
* entityManager.getReference() 延迟加载
*
*/
@Test
@Transactional
public void testGetOne(){
Customer customer = customerDao.getOne(4L);
System.out.println(customer);
}
}
<file_sep>/common-jpa/src/test/java/com/phoenix/test/JpaTest.java
package com.phoenix.test;
import com.phoenix.pojo.Customer;
import com.phoenix.util.JpaUtil;
import org.junit.Test;
import javax.persistence.EntityManager;
import javax.persistence.EntityManagerFactory;
import javax.persistence.EntityTransaction;
import javax.persistence.Persistence;
public class JpaTest {
/**
* 测试jpa的保存
* jpa的操作步骤
* 1.加载配置文件创建工厂(实体管理器工厂)对象
* 2.通过实体管理器工厂获取实体管理器
* 3.获取事物对象,开启事务
* 4.完成增删改查操作
* 5.提交事务(回滚事务)
* 6.释放资源
*/
@Test
public void testSave(){
//1.加载配置文件创建工厂(实体管理器工厂)对象
//persistenceUnitName 就是persistence.xml中配置的name
EntityManagerFactory entityManagerFactory = Persistence.createEntityManagerFactory("myJpa");
//2.通过实体管理器工厂获取实体管理器
EntityManager entityManager = entityManagerFactory.createEntityManager();
//3.获取事物对象,开启事务
EntityTransaction transaction = entityManager.getTransaction();
transaction.begin();
Customer customer=new Customer();
customer.setCustName("tomcat");
customer.setCustIndustry("it");
//4.完成增删改查操作
entityManager.persist(customer);//保存操作
//5.提交事务(回滚事务)
transaction.commit();
//6.释放资源
entityManager.close();
entityManagerFactory.close();
}
@Test
public void testSaveByUtil(){
//1.通过工具类获取entityManager对象
EntityManager entityManager = JpaUtil.getEntityManager();
//2.获取事物对象,开启事务
EntityTransaction transaction = entityManager.getTransaction();
transaction.begin();
Customer customer=new Customer();
customer.setCustName("tomcat2");
customer.setCustIndustry("it2");
//3.完成增删改查操作
entityManager.persist(customer);//保存操作
//4.提交事务(回滚事务)
transaction.commit();
//5.释放资源
entityManager.close();
}
/**
* find:(立即加载)
* 立即发送sql进行查询
*/
@Test
public void testFindById(){
//1.通过工具类获取entityManager对象
EntityManager entityManager = JpaUtil.getEntityManager();
//2.获取事物对象,开启事务
EntityTransaction transaction = entityManager.getTransaction();
transaction.begin();
/**
* find 根据id查询数据
* class查询数据的结果需要包装的实体类类型的字节码
* id 查询的主键
*/
Customer customer = entityManager.find(Customer.class, 2L);
System.out.println(customer);
//4.提交事务(回滚事务)
transaction.commit();
//5.释放资源
entityManager.close();
}
/**
* getReference: (延时加载)
* 1.获得的对象是一个动态代理对象
* 2.调用getReference不会立即发送sql语句查询数据库
* 当调用结果对象的时候,才会发送sql进行查询
*/
@Test
public void testReferenceById(){
//1.通过工具类获取entityManager对象
EntityManager entityManager = JpaUtil.getEntityManager();
//2.获取事物对象,开启事务
EntityTransaction transaction = entityManager.getTransaction();
transaction.begin();
/**
* find 根据id查询数据
* class查询数据的结果需要包装的实体类类型的字节码
* id 查询的主键
*/
Customer customer = entityManager.getReference(Customer.class, 2L);
System.out.println(customer);
//4.提交事务(回滚事务)
transaction.commit();
//5.释放资源
entityManager.close();
}
/**
* jpa更新数据
*/
@Test
public void testUpdate(){
//1.通过工具类获取entityManager对象
EntityManager entityManager = JpaUtil.getEntityManager();
//2.获取事物对象,开启事务
EntityTransaction transaction = entityManager.getTransaction();
transaction.begin();
Customer customer = entityManager.find(Customer.class, 2L);
customer.setCustName("merge2");
Customer merge = entityManager.merge(customer);
System.out.println(merge);
//4.提交事务(回滚事务)
transaction.commit();
//5.释放资源
entityManager.close();
}
/**
* jpa删除数据
*/
@Test
public void testRemove(){
//1.通过工具类获取entityManager对象
EntityManager entityManager = JpaUtil.getEntityManager();
//2.获取事物对象,开启事务
EntityTransaction transaction = entityManager.getTransaction();
transaction.begin();
Customer customer = entityManager.find(Customer.class, 1L);
entityManager.remove(customer);
//4.提交事务(回滚事务)
transaction.commit();
//5.释放资源
entityManager.close();
}
}
<file_sep>/README.md
## jpa
## jpa规范

## springData jpa规范
### springDataJpa jpa hibernate关系

<file_sep>/spring-data-jpa/src/test/java/com/phoenix/test/CustomerJpqlTest.java
package com.phoenix.test;
import com.phoenix.dao.CustomerDao;
import com.phoenix.domain.Customer;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.annotation.Rollback;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import org.springframework.transaction.annotation.Transactional;
@RunWith(SpringJUnit4ClassRunner.class) //声明spring提供的单元测试环境
@ContextConfiguration(locations = "classpath:applicationContext.xml") //指定spring容器的配置信息
public class CustomerJpqlTest {
@Autowired
private CustomerDao customerDao;
@Test
public void testFindByNameJpql(){
Customer customer = customerDao.findByNameByJpql("tomcat4");
System.out.println(customer);
}
@Test
public void testFindByNameAndIdJpql(){
Customer customer = customerDao.findByNameAndIdByJpql("tomcat4",4L);
System.out.println(customer);
}
/**
* springdata-jpa中使用update更新操作,
* 1.需要手动添加事务 @transactional
* 2.并且默认会自动回滚,需要手动添加 @rollback(value=false)
*
*/
@Test
@Transactional //添加事务支持
@Rollback(value = false)
public void testUpdate(){
customerDao.updateCustomerName("update by jpql",4L);
}
}
| aea2ad1e163d6d862cfe927b42c2a7de1da68210 | [
"Markdown",
"Java"
] | 6 | Java | PhoenixFate/darkhorse297-jpa | bdf86ec51846ead2556927c955eef37fd1046bdf | a62956aedc1ca154e30a9e91dc7c4a07bb03b175 | |
refs/heads/main | <repo_name>mattatti/MineCraft-ripoff<file_sep>/Assets/Scripts/Chunk.cs
using System.Collections;
using System.Collections.Generic;
using System.Collections.Specialized;
using UnityEngine;
public class Chunk {
public ChunkCoord coord;
private GameObject chunkObject;
private MeshRenderer meshRenderer;
private MeshFilter meshFilter;
private int vertexIndex = 0;
private readonly List<Vector3> vertices = new List<Vector3>();
private readonly List<int> triangles = new List<int>();
private readonly List<Vector2> uvs = new List<Vector2>();
private byte[,,] voxelMap = new byte[VoxelData.ChunkWidth, VoxelData.ChunkHeight, VoxelData.ChunkWidth];
private readonly World world;
public Chunk(ChunkCoord _coord, World _world)
{
coord = _coord;
world = _world;
chunkObject = new GameObject();
meshFilter = chunkObject.AddComponent<MeshFilter>();
meshRenderer = chunkObject.AddComponent<MeshRenderer>();
meshRenderer.material = world.material;
chunkObject.transform.SetParent(world.transform);
chunkObject.transform.position = new Vector3(coord.x * VoxelData.ChunkWidth, 0f, coord.z * VoxelData.ChunkWidth);
chunkObject.name = "Chunk " + coord.x + ", " + coord.z;
PopulateVoxelMap();
CreateMeshData();
CreateMesh();
}
void PopulateVoxelMap() {
for (var y = 0; y < VoxelData.ChunkHeight; y++) {
for (var x = 0; x < VoxelData.ChunkWidth; x++) {
for (var z = 0; z < VoxelData.ChunkWidth; z++) {
voxelMap[x, y, z] = world.GetVoxel(new Vector3(x, y, z) + Position);
}
}
}
}
void CreateMeshData() {
for (var y = 0; y < VoxelData.ChunkHeight; y++)
{
for (var x = 0; x < VoxelData.ChunkWidth; x++)
{
for (var z = 0; z < VoxelData.ChunkWidth; z++)
{
if(world.blockTypes[voxelMap[x,y,z]].isSolid)
AddVoxelDataToChunk(new Vector3(x, y, z));
}
}
}
}
public bool IsActive
{
get { return chunkObject.activeSelf; }
set { chunkObject.SetActive(value); }
}
public Vector3 Position
{
get { return chunkObject.transform.position; }
}
bool IsVoxelInChunk(int x,int y, int z)
{
if (x < 0 || x > VoxelData.ChunkWidth - 1 || y < 0 || y > VoxelData.ChunkHeight - 1 || z < 0 || z > VoxelData.ChunkWidth - 1)
return false;
else
return true;
}
bool CheckVoxel(Vector3 pos)
{
int x = Mathf.FloorToInt(pos.x);
int y = Mathf.FloorToInt(pos.y);
int z = Mathf.FloorToInt(pos.z);
if (!IsVoxelInChunk(x,y,z) )
return world.blockTypes[world.GetVoxel(pos + Position)].isSolid;
return world.blockTypes[voxelMap[x, y, z]].isSolid;
}
void AddVoxelDataToChunk(Vector3 pos) {
for (int p = 0; p < 6; p++) {
if (!CheckVoxel(pos + VoxelData.faceChecks[p]))
{
byte blockID = voxelMap[(int)pos.x, (int)pos.y, (int)pos.z];
vertices.Add(pos + VoxelData.voxelVerts[VoxelData.voxelTris[p, 0]]);
vertices.Add(pos + VoxelData.voxelVerts[VoxelData.voxelTris[p, 1]]);
vertices.Add(pos + VoxelData.voxelVerts[VoxelData.voxelTris[p, 2]]);
vertices.Add(pos + VoxelData.voxelVerts[VoxelData.voxelTris[p, 3]]);
AddTexture(world.blockTypes[blockID].GetTextureID(p));
triangles.Add(vertexIndex);
triangles.Add(vertexIndex+1);
triangles.Add(vertexIndex+2);
triangles.Add(vertexIndex+2);
triangles.Add(vertexIndex+1);
triangles.Add(vertexIndex+3);
vertexIndex += 4;
}
}
}
void CreateMesh()
{
Mesh mesh = new Mesh
{
vertices = vertices.ToArray(),
triangles = triangles.ToArray(),
uv = uvs.ToArray()
};
mesh.RecalculateNormals();
meshFilter.mesh = mesh;
}
void AddTexture(int textureID)
{
float y = textureID / VoxelData.TextureAtlasSizeInBlocks;
float x = textureID - y * VoxelData.TextureAtlasSizeInBlocks;
x *= VoxelData.NormalizeBlockTextureSize;
y *= VoxelData.NormalizeBlockTextureSize;
y = 1f - y - VoxelData.NormalizeBlockTextureSize;
uvs.Add(new Vector2(x, y));
uvs.Add(new Vector2(x, y + VoxelData.NormalizeBlockTextureSize));
uvs.Add(new Vector2(x + VoxelData.NormalizeBlockTextureSize, y));
uvs.Add(new Vector2(x + VoxelData.NormalizeBlockTextureSize, y + VoxelData.NormalizeBlockTextureSize));
}
}
public class ChunkCoord
{
public int x;
public int z;
public ChunkCoord(int _x, int _z)
{
x = _x;
z = _z;
}
public bool Equals(ChunkCoord other)
{
if (other == null)
return false;
else if (other.x == x && other.z == z)
return true;
else return false;
}
}
<file_sep>/Assets/Scripts/World.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class World : MonoBehaviour
{
public int seed;
public Transform player;
public Vector3 spawnPosition;
public Material material;
public BlockType[] blockTypes;
public Chunk[,] chunks = new Chunk[VoxelData.WorldSizeInChunks,VoxelData.WorldSizeInChunks];
private List<ChunkCoord> activeChunks = new List<ChunkCoord>();
private ChunkCoord playerChunkCoord;
private ChunkCoord playerLastChunkCoord;
private void Start()
{
Random.InitState(seed);
spawnPosition = new Vector3((VoxelData.WorldSizeInChunks * VoxelData.ChunkWidth) / 2f, VoxelData.ChunkHeight +2, (VoxelData.WorldSizeInChunks * VoxelData.ChunkWidth) / 2f);
GenerateWorld();
playerLastChunkCoord = GetChunkCoordFromVector3(player.position);
}
private void Update()
{
playerChunkCoord = GetChunkCoordFromVector3(player.position);
if(!playerChunkCoord.Equals(playerLastChunkCoord))
CheckViewDistance();
}
public byte GetVoxel(Vector3 pos)
{
int yPos = Mathf.FloorToInt(pos.y);
/*IMMUTABLE PASS*/
//if outside World, return air.
if (!IsVoxelInWorld(pos))
return 0;
//if bottom block of chunk, return bedrock.
if (yPos == 0)
return 1;
/* BASIC TERRAIN PASS */
int terrainHeight = Mathf.FloorToInt(VoxelData.ChunkHeight * Noise.Get2DPerlin(new Vector2(pos.x, pos.z),500, 0.25f));
if (yPos <= terrainHeight)
return 3;
else
return 0;
}
private void GenerateWorld()
{
for(var x =(VoxelData.WorldSizeInChunks / 2) - VoxelData.ViewDistranceInChunks; x < (VoxelData.WorldSizeInChunks / 2) + VoxelData.ViewDistranceInChunks; x++)
{
for (var z = (VoxelData.WorldSizeInChunks / 2) - VoxelData.ViewDistranceInChunks; z < (VoxelData.WorldSizeInChunks / 2) + VoxelData.ViewDistranceInChunks; z++)
{
CreateNewChunk(x, z);
}
}
player.position = spawnPosition;
}
ChunkCoord GetChunkCoordFromVector3(Vector3 pos)
{
int x = Mathf.FloorToInt(pos.x / VoxelData.ChunkWidth);
int z = Mathf.FloorToInt(pos.z / VoxelData.ChunkWidth);
return new ChunkCoord(x, z);
}
void CheckViewDistance()
{
ChunkCoord coord = GetChunkCoordFromVector3(player.position);
List<ChunkCoord> previouslyActiveChunks = new List<ChunkCoord>(activeChunks);
for (var x = coord.x - VoxelData.ViewDistranceInChunks; x < coord.x + VoxelData.ViewDistranceInChunks; x++)
{
for (var z = coord.z - VoxelData.ViewDistranceInChunks; z < coord.z + VoxelData.ViewDistranceInChunks; z++)
{
if(IsChunkInWorld(new ChunkCoord(x,z)))
{
if (chunks[x, z] == null)
CreateNewChunk(x,z);
else if(!chunks[x,z].IsActive)
{
chunks[x, z].IsActive = true;
activeChunks.Add(new ChunkCoord(x, z));
}
}
for(var i=0; i < previouslyActiveChunks.Count; i++)
{
if (previouslyActiveChunks[i].Equals(new ChunkCoord(x, z)))
previouslyActiveChunks.RemoveAt(i);
}
}
}
foreach(var c in previouslyActiveChunks)
{
chunks[c.x, c.z].IsActive = false;
}
}
private void CreateNewChunk(int x, int z)
{
chunks[x, z] = new Chunk(new ChunkCoord(x, z), this);
activeChunks.Add(new ChunkCoord(x,z));
}
bool IsChunkInWorld(ChunkCoord coord)
{
if (coord.x > 0 && coord.x < VoxelData.WorldSizeInChunks - 1 && coord.z > 0 && coord.z < VoxelData.WorldSizeInChunks - 1)
return true;
else
return false;
}
bool IsVoxelInWorld(Vector3 pos)
{
if (pos.x >= 0 && pos.x < VoxelData.WorldSizeInVoxels && pos.y >= 0 && pos.y < VoxelData.ChunkHeight && pos.z >= 0 && pos.z < VoxelData.WorldSizeInVoxels)
return true;
else
return false;
}
}
[System.Serializable]
public class BlockType
{
public string blockName;
public bool isSolid;
[Header("Texture Values")]
public int backFaceTexute;
public int frontFaceTexute;
public int topFaceTexute;
public int bottomFaceTexute;
public int leftFaceTexute;
public int rightFaceTexute;
//Back, Front, Top, Bottom, Left, Right
public int GetTextureID(int faceIndex)
{
switch (faceIndex)
{
case 0:
return backFaceTexute;
case 1:
return frontFaceTexute;
case 2:
return topFaceTexute;
case 3:
return bottomFaceTexute;
case 4:
return leftFaceTexute;
case 5:
return rightFaceTexute;
default:
Debug.Log("Error in GetTextureID; invalid face index");
return 0;
}
}
}
| a2826476a93aab092ab80ba1c05d8074f49b3901 | [
"C#"
] | 2 | C# | mattatti/MineCraft-ripoff | e0d7b718e096331630f3b572c913e593c49594f2 | c8c8268425b7e211b8d60421fece4747f980bf1c | |
refs/heads/master | <repo_name>ckopecky/Prerequisite-Coursework<file_sep>/README.md
# Prerequisite-Coursework
Prerequisite Coursework for Lambda School
<file_sep>/Udacity Javascript Course Materials.js
// Define two variables called thingOne and thingTwo and assign them values. Print the values of both variables in one console.log statement using concatenation. For example,
// red blue where "red" is the value of thingOne and "blue" is the value of thingTwo. Don't forget to use semicolons!
/*
* Programming Quiz: Semicolons! (2-8)
*/
var thingOne = "red";
var thingTwo = "blue";
console.log(thingOne + thingTwo);
// Programming Quiz: What's my Name? (2-9)
// Directions: Create a variable called fullName and assign it your full name as a string.
var fullName = "<NAME>";
console.log (fullName);
/*
* Programming Quiz: Out to Dinner (2-10)
*/
// Create a variable called bill and assign it the result of 10.25 + 3.99 + 7.15 (don't perform the
// calculation yourself, let JavaScript do it!). Next, create a variable called tip and assign it the
// result of multiplying bill by a 15% tip rate. Finally, add the bill and tip together and store it
// into a variable called total.
// Print the total to the JavaScript console.
// Hint: 15% in decimal form is written as 0.15.
var bill = (10.25 +3.99+7.15);
var tip = bill*.15;
var total = bill + tip;
console.log('$'+total.toFixed(2));
//Programming Quiz: MadLibs 2-11
// Directions:
// Mad Libs is a word game where players have fun substituting words for blanks in a story. For this exercise, use the adjective variables below to fill in the blanks and complete the following message.
// "The Intro to JavaScript course is __________. James and Julia are so __________. I cannot wait to work through the rest of this __________ content!"
// var adjective1 = "amazing";
// var adjective2 = "fun";
// var adjective3 = "entertaining";
// Assign the resulting string to a variable called madLib.
var adjective1 = 'amazing';
var adjective2 = 'fun';
var adjective3 = 'entertaining';
var madLib = 'The Intro to JavaScript course is '+adjective1+'. James and Julia are so '+adjective2+'. I cannot wait to work through the rest of this '+adjective3+' content!';
console.log (madLib);
/*
* Programming Quiz: One Awesome Message (2-12)
*
* 1. Create the variables:
* - firstName
* - interest
* - hobby
* 2. Create a variable named awesomeMessage and, using string concatenation
and the variables above, create an awesome message.
* 3. Print the awesomeMessage variable to the console.
*/
/*
* Notes:
* - Using the above as an example, firstName would have been assigned to
* "Julia", interest to "cats", and hobby to "play video games".
* - Be sure to include spaces and periods where necessary!
*/
var firstName = "Christina";
var interest = "cats";
var hobby = "play my flute";
var awesomeMessage ="Hi, my name is "+firstName+". I love "+interest+". In my spare time, I like to "+hobby+".";
console.log (awesomeMessage);
/*
* Programming Quiz: Even or Odd (3-2)
*
* Write an if...else statement that prints `even` if the
* number is even and prints `odd` if the number is odd.
*
* Note - make sure to print only the string "even" or the string "odd"
*/
// change the value of `number` to test your if...else statement
var number = 3;
if (number%2===0) {
console.log('even');
} else {
console.log ('odd');
}
/*
* Programming Quiz: Musical Groups (3-3)
prints "not a group" if musicians is less than or equal to 0
prints "solo" if musicians is equal to 1
prints "duet" if musicians is equal to 2
prints "trio" if musicians is equal to 3
prints "quartet" if musicians is equal to 4
prints "this is a large group" if musicians is greater than 4
*/
// change the value of `musicians` to test your conditional statements
var musicians = 0;
if (musicians>4){
console.log('this is a large group');
}
else if (musicians == 4){
console.log("quartet");
}
else if (musicians == 3){
console.log("trio");
}
else if (musicians == 2){
console.log("duet");
}
else if (musicians == 1){
console.log("solo");
}
else{
console.log("not a group");
}
//Programming quiz 3-4 - fictitious murder mystery
// change the value of `room` and `suspect` to test your code
var room = "gallery";
var suspect = "<NAME>";
var weapon = "";
var solved = false;
if (room === "gallery") {
weapon = "trophy";
if (suspect === "<NAME>") {
solved = true;
}
} else if (room === "ballroom") {
weapon = "poison";
if (suspect === "Mr. Kalehoff") {
solved = true;
}
} else if (room === "billiards room") {
weapon = "pool stick";
if (suspect === "<NAME>") {
solved = true;
}
} else {
weapon = "knife";
if (suspect === "Mr. Parkes") {
solved = true;
}
}
if (solved) {
console.log(suspect +" did it in the "+ room + " with the "+ weapon +"!");
}
/*
* Programming Quiz - Checking Your Balance (3-5)
*/
// change the values of `balance`, `checkBalance`, and `isActive` to test your code
var balance = 0;
var checkBalance = true;
var isActive = true;
// your code goes here
if (checkBalance === true) {
if ((balance > 0) && (isActive === true)) {
console.log("Your balance is $" + balance.toFixed(2)+".");
}
else if (isActive === false) {
console.log("Your account is no longer active.");
}
else if (balance === 0) {
console.log("Your account is empty.");
}
else {
console.log("Your balance is negative. Please contact bank.");
}
}
else {
console.log("Thank you. Have a nice day!");
}
/*
* Programming Quiz: Ice Cream (3-6)
*
* Write a single if statement that logs out the message:
*
* "I'd like two scoops of __________ ice cream in a __________ with __________."
*
* ...only if:
* - flavor is "vanilla" or "chocolate"
* - vessel is "cone" or "bowl"
* - toppings is "sprinkles" or "peanuts"
*
* We're only testing the if statement and your boolean operators.
* It's okay if the output string doesn't match exactly.
*/
// change the values of `flavor`, `vessel`, and `toppings` to test your code
var flavor = "chocolate";
var vessel = "cone";
var toppings = "sprinkles";
if ((flavor === "vanilla"||flavor === "chocolate") && (vessel ==="cone"||vessel==="bowl") &&
(toppings==="sprinkles"||toppings==="peanuts")){
console.log ("I\'d like two scoops of "+flavor+" ice cream in a "+ vessel+" with "+toppings+".");}
/*
* Programming Quiz: What do I Wear? (3-7)
*/
// change the values of `shirtWidth`, `shirtLength`, and `shirtSleeve` to test your code
var shirtWidth = 0;
var shirtLength = 0;
var shirtSleeve = 0;
if((shirtWidth <= 18) && (shirtLength <= 28) && (shirtSleeve <= 8.13)) {
console.log("S");
}
else if((shirtWidth <= 20 && shirtWidth > 18) && (shirtLength <= 29 && shirtLength > 28) && (shirtSleeve <= 8.38 && shirtSleeve > 8.13)) {
console.log("M");
}
else if((shirtWidth <= 22 && shirtWidth > 20) && (shirtLength <= 30 && shirtLength > 29) && (shirtSleeve <= 8.63 && shirtSleeve > 8.38)) {
console.log("L");
}
else if((shirtWidth <= 24 && shirtWidth > 22) && (shirtLength <= 31 && shirtLength > 30) && (shirtSleeve <= 8.88 && shirtSleeve > 8.63)) {
console.log("XL");
}
else if((shirtWidth <= 26 && shirtWidth > 24) && (shirtLength <= 33 && shirtLength > 31) && (shirtSleeve <= 9.63 && shirtSleeve > 8.88)) {
console.log("2XL");
}
else if((shirtWidth <= 28 && shirtWidth > 26) && (shirtLength <= 34 && shirtLength > 33) && (shirtSleeve <= 10.13 && shirtSleeve > 9.63)) {
console.log("3XL");
}
else {
console.log("N/A");
}
/*
* Programming Quiz - Navigating the Food Chain (3-8)
*
* Use a series of ternary operator to set the category to one of the following:
* - "herbivore" if an animal eats plants
* - "carnivore" if an animal eats animals
* - "omnivore" if an animal eats plants and animals
* - undefined if an animal doesn't eat plants or animals
*
* Notes
* - use the variables `eatsPlants` and `eatsAnimals` in your ternary expressions
* - `if` statements aren't allowed ;-)
*/
// change the values of `eatsPlants` and `eatsAnimals` to test your code
var eatsPlants = true;
var eatsAnimals = false;
var category = eatsPlants&&eatsAnimals? "omnivore":eatsPlants? "herbivore": eatsAnimals? "carnivore":undefined;
console.log(category);
/*
* Programming Quiz: Back to School (3-9)
*/
//education is the object of the switch statement and the salarty will be the output
// change the value of `education` to test your code
var education = "no high school diploma";
// set the value of this based on a person's education
var salary;
switch(education){
case "no high school diploma":
salary = "$"+25+','+636;
console.log ('In 2015, a person with '+education+' earned an average of '+ salary+'/year.');
break;
case "a high school diploma":
salary = "$"+35+','+256;
console.log ('In 2015, a person with '+education+' earned an average of '+ salary+'/year.');
break;
case "an Associate's degree":
salary = "$"+41+','+496;
console.log ('In 2015, a person with '+education+' earned an average of '+ salary+'/year.');
break;
case "a Bachelor's degree":
salary = "$"+59+','+124;
console.log ('In 2015, a person with '+education+' earned an average of '+ salary+'/year.');
break;
case "a Master's degree":
salary = "$"+69+','+732;
console.log ('In 2015, a person with '+education+' earned an average of '+ salary+'/year.');
break;
case "a Professional degree":
salary = "$"+89+','+960;
console.log ('In 2015, a person with '+education+' earned an average of '+ salary+'/year.');
break;
case "a Doctoral degree":
salary = "$"+84+','+396;
console.log ('In 2015, a person with '+education+' earned an average of '+ salary+'/year.');
break;
}
/*
* Programming Quiz: Changing the Loop (4-4)
*/
// rewrite the while loop as a for loop
// var x = 9;
// while (x >= 1) {
// console.log("hello " + x);
// x = x - 1;
// }
for(let x=9;x>=1;x--){
console.log("hello "+ x);
}
/*
// * Programming Quiz: Fix the Error 1 (4-5)
// */
// for (x < 10; x++) {
// console.log(x);
// }
// fix the for loop
for (let x=5; x < 10; x++) {
console.log(x);
}
/*
* Programming Quiz: Fix the Error 2 (4-6)
*/
// fix the for loop
//for (var k = 0 k < 200 k++) {
// console.log(k);
//}
for (var k = 0; k < 200; k++) {
console.log(k);
}
/*
* Programming Quiz: Factorials (4-7)
*/
let solution =1;
for (let inputNumber =1; inputNumber<=12;inputNumber++){
solution *=inputNumber;
}
console.log(solution);
/*
* Programming Quiz: Find my Seat (4-8)
*
* Write a nested for loop to print out all of the different seat combinations in the theater.
* The first row-seat combination should be 0-0
* The last row-seat combination will be 25-99
*
* Things to note:
* - the row and seat numbers start at 0, not 1
* - the highest seat number is 99, not 100
*/
for (let x = 0; x<26;x++){
for(let y = 0; y<100;y++){
console.log(x+'-'+y);
}
}
/*
* Programming Quiz: Laugh it Off 1 (5-1)
*/
function laugh(){
return "hahahahahahahahahaha!";
}
console.log(laugh());
/*
* Programming Quiz: Laugh it Off 2 (5-2)
*
* Write a function called `laugh` with a parameter named `num` that represents the number of "ha"s to return.
*
*
Note:
* - make sure your the final character is an exclamation mark ("!")
* - make sure that your function produces the correct results when it is called multiple times
*/
function laugh(num){
let ha="";
for (let i = 0;i<num;i++){
ha+= "ha";
}
return ha+"!";
}
console.log(laugh(3));
/*
* Programming Quiz: Build A Triangle (5-3)
*/
// creates a line of * for a given length
function makeLine(length) {
var line = "";
for (var j = 1; j <= length; j++) {
line += "* ";
}
return line + "\n";
}
function buildTriangle(width){
var triangle = '';
for (i=1;i<=width;i++){
triangle += (makeLine(i));
// console.log (triangle);
}
return triangle;
}
// test your code by uncommenting the following line
console.log(buildTriangle(10));
/*
* Programming Quiz: Laugh (5-4)
*/
var laugh = function (num){
let ha='';
for (let i = 0;i<num;i++){
ha+= "ha";
}
return ha+"!";
};
console.log(laugh(10));
/*
* Programming Quiz: Cry (5-5)
*/
var x= function cry(boohoo){
return boohoo+ '!';
};
{
return x;
}
cry(boohoo);
/*
* Programming Quiz: Inline Functions (5-6)
*/
// don't change this code
function emotions(myString, myFunc) {
console.log("I am " + myString + ", " + myFunc(2));
}
// your code goes here
emotions("happy", function laugh(num){
var ha = "";
for (x = 0; x < num; x++){
ha += "ha";
}
return ha + "!";
});
/*
* Programming Quiz: UdaciFamily (6-1)
*/
var udaciFamily = ["Julia", "James", "Christina"];
console.log (udaciFamily);
/*
* Programming Quiz: Building the Crew (6-2)
*/
var captain = "Mal";
var second = "Zoe";
var pilot = "Wash";
var companion = "Inara";
var mercenary = "Jayne";
var mechanic = "Kaylee";
var crew = [captain, second, pilot, companion, mercenary, mechanic];
console.log (crew);
/*
* Programming Quiz: The Price is Right (6-3)
*/
var prices = [1.23, 48.11, 90.11, 8.50, 9.99, 1.00, 1.10, 67.00];
prices[0]=2.25;
prices[2]=100.65;
prices[6]=2.25;
console.log (prices);
/*
* Programming Quiz: Colors of the Rainbow (6-4)
*/
var rainbow = ["Red", "Orange", "Blackberry", "Blue"];
rainbow.splice(-2, 1, "Yellow", "Green");
rainbow.splice(5, 0, "Purple");
console.log (rainbow);
/*
* Programming Quiz: Quidditch Cup (6-5)
*/
var team = ["<NAME>", "<NAME>", "<NAME>", "<NAME>", "<NAME>", "<NAME>", "<NAME>"];
function hasEnoughPlayers(team){
var teamLength = team.length;
if(teamLength >= 7){
return true;
}else{
return false;
}
}
console.log(hasEnoughPlayers(team));
/*
* Programming Quiz: Joining the Crew (6-6)
*/
var captain = "Mal";
var second = "Zoe";
var pilot = "Wash";
var companion = "Inara";
var mercenary = "Jayne";
var mechanic = "Kaylee";
var crew = [captain, second, pilot, companion, mercenary, mechanic];
var doctor = "Simon";
var sister = "River";
var shepherd = "Book";
crew.push (doctor, sister, shepherd);
console.log (crew);
/*
* Programming Quiz: Another Type of Loop (6-8)
*
* Use the existing `test` variable and write a `forEach` loop
* that adds 100 to each number that is divisible by 3.
*
* Things to note:
* - you must use an `if` statement to verify code is divisible by 3
* - you can use `console.log` to verify the `test` variable when you're finished looping
*/
var test = [12, 929, 11, 3, 199, 1000, 7, 1, 24, 37, 4,
19, 300, 3775, 299, 36, 209, 148, 169, 299,
6, 109, 20, 58, 139, 59, 3, 1, 139
];
// Write your code here
test.forEach(function(num, index, array){
if(num % 3 === 0){
array[index] = num += 100;
}
});
console.log(test);
/*
* Programming Quiz: I Got Bills (6-9)
*
* Use the .map() method to take the bills array below and create a second array
* of numbers called totals. The totals array should contains each amount from the
* bills array but with a 15% tip added. Log the totals array to the console.
*
* For example, the first two entries in the totals array would be:
*
* [57.76, 21.99, ... ]
*
* Things to note:
* - each entry in the totals array must be a number
* - each number must have an accuracy of two decimal points
*/
var bills = [50.23, 19.12, 34.01,
100.11, 12.15, 9.90, 29.11, 12.99,
10.00, 99.22, 102.20, 100.10, 6.77, 2.22
];
var totals = bills.map(function(num){
num = Number(((num * 0.15)+num).toFixed(2));
return num;
});
console.log (totals);
/*
* Programming Quiz: Nested Numbers (6-10)
*
* - The `numbers` variable is an array of arrays.
* - Use a nested `for` loop to cycle through `numbers`.
* - Convert each even number to the string "even"
* - Convert each odd number to the string "odd"
*/
var numbers = [
[243, 12, 23, 12, 45, 45, 78, 66, 223, 3],
[34, 2, 1, 553, 23, 4, 66, 23, 4, 55],
[67, 56, 45, 553, 44, 55, 5, 428, 452, 3],
[12, 31, 55, 445, 79, 44, 674, 224, 4, 21],
[4, 2, 3, 52, 13, 51, 44, 1, 67, 5],
[5, 65, 4, 5, 5, 6, 5, 43, 23, 4424],
[74, 532, 6, 7, 35, 17, 89, 43, 43, 66],
[53, 6, 89, 10, 23, 52, 111, 44, 109, 80],
[67, 6, 53, 537, 2, 168, 16, 2, 1, 8],
[76, 7, 9, 6, 3, 73, 77, 100, 56, 100]
];
for(var row = 0; row < numbers.length; row++){
for(var column = 0; column < numbers[row].length; column++){
numbers[row][column] = numbers[row][column] % 2 === 0 ? "even" : "odd";
}
}
console.log(numbers);
//Programming Quiz 7-1
var umbrella = {
color: "pink",
isOpen: false,
open: function() {
if (umbrella.isOpen === true) {
return "The umbrella is already opened!";
} else {
umbrella.isOpen = true;
return "Julia opens the umbrella!";
}
},
isClose: true,
close: function () {
if (umbrella.isClosed === true) {
return "The umbrella is already closed!";
}else {
umbrella.isClosed = true;
umbrella.isOpen = false;
return "Julia closes the umbrella";
}
},
};
/*
* Programming Quiz: Menu Items (7-2)
*/
let breakfast = {
name: "<NAME>",
price: 9.95,
ingredients: ["eggs", "sausage", "toast", "hashbrowns", "pancakes"],
};
console.log (breakfast);
/*
* Programming Quiz: Bank Accounts 1 (7-3)
*/
var savingsAccount = {
balance: 1000,
interestRatePercent: 1,
deposit: function addMoney(amount) {
if (amount > 0) {
savingsAccount.balance += amount;
}
},
withdraw: function removeMoney(amount) {
var verifyBalance = savingsAccount.balance - amount;
if (amount > 0 && verifyBalance >= 0) {
savingsAccount.balance -= amount;
}
},
printAccountSummary: function print(balance, interestRatePercent){
return "Welcome!\nYour balance is currently $"+ savingsAccount.balance+ " and your interest rate is "+ savingsAccount.interestRatePercent+"%.";
}
};
console.log(savingsAccount.printAccountSummary());
/*
* Programming Quiz: Facebook Friends (7-5)
*/
var facebookProfile = {
name: "Christina",
friends: 423,
messages: ["Hi! How are you?", "Happy Birthday!", "Congratulations!"],
postMessage: function postMessage(message){
var newMessage = '';
facebookProfile.messages.push(newMessage);
return facebookProfile.messages;
},
deleteMessage: function deleteMessage(index){
facebookProfile.messages.splice(index, 1);
return facebookProfile.messages;
},
addFriend: function addFriend(){
facebookProfile.friends=facebookProfile.friends+1;
return facebookProfile.friends;
},
removeFriend: function removeFriend(){
facebookProfile.friends = facebookProfile.friends -1;
return facebookProfile.friends;
}
};
console.log(facebookProfile);
/*
* Programming Quiz: Donuts Revisited (7-6)
*/
var donuts = [
{ type: "Jelly", cost: 1.22 },
{ type: "Chocolate", cost: 2.45 },
{ type: "Cider", cost: 1.59 },
{ type: "Boston Cream", cost: 5.99 }
];
donuts.forEach(function(donuts) {
console.log(donuts.type +" donuts cost $"+ donuts.cost + " each");
});
| cdff72478979877cdb7c968daa0633fb440f0bd6 | [
"Markdown",
"JavaScript"
] | 2 | Markdown | ckopecky/Prerequisite-Coursework | 8b988b5acb76d0fff1acc7708eb2c96020badb75 | 1e50e03c831369ebe04f64906dfffb0b104b29d0 | |
refs/heads/main | <repo_name>awakoto384/Photography-portfolio<file_sep>/src/pages/AboutPage.js
import React from "react";
import Hero from "../components/Hero";
import Content from "../components/Content";
function AboutPage(props) {
return (
<div>
<Hero title={props.title} />
<Content>
<p>
Hello, my name is <NAME>. I'm a full-time student who is majoring
in web design :){" "}
</p>
<p>
I designed this website for displaying my photo essay. I've been
taught myself photography for more than three years, since I got my
first camera as the birthday gift from my parents.
</p>
<p>
I mainly focus on still life photography and portrait. Making simple
things look stunning is always what I really enjoy..{" "}
</p>
<p>
My dream is to become a software engineer and one day start my own
photography studio as a side job.{" "}
</p>
<p>
In the future, I will strive to create better works. Hope you enjoy my
website!
</p>
</Content>
</div>
);
}
export default AboutPage;
| 5cf3bb8d9a70df13f45718ad9bbe9ce58bc1b28a | [
"JavaScript"
] | 1 | JavaScript | awakoto384/Photography-portfolio | 52ef5714f06146641c9c3450ced191d45003366e | 5276202796178477e9f0dc6ca6f36816f0d009be | |
refs/heads/master | <file_sep>#ifndef SUPERFICIE_H
#define SUPERFICIE_H
#include "vertice.h"
class Superficie {
private:
Vertice *inicio;
Vertice *final;
Superficie *sig;
float rojo, verde, azul;
float nx, ny, nz;
public:
Superficie();
~Superficie();
Superficie *getSig();
void setSig(Superficie *);
void setColor(float, float, float);
void setNormal(float, float, float);
Superficie *copia();
void agregar(Vertice *);
void transformar(Matriz3D *);
void desplegar();
};
#endif // SUPERFICIE_H
<file_sep>#include "pantalla.h"
Pantalla::Pantalla() : Objeto3D() {
}
Eje *Pantalla::getEjeVer() {
return ejeVer;
}
Eje *Pantalla::getEjeHor() {
return ejeHor;
}
void Pantalla::setEjeVer(Eje *ejeVer) {
this->ejeVer = ejeVer;
}
void Pantalla::setEjeVer(float x1, float y1, float z1, float x2, float y2, float z2) {
ejeVer = new Eje(new Punto(x1, y1, z1), new Punto(x2, y2, z2));
}
void Pantalla::setEjeHor(Eje *ejeHor) {
this->ejeHor = ejeHor;
}
void Pantalla::setEjeHor(float x1, float y1, float z1, float x2, float y2, float z2) {
ejeHor = new Eje(new Punto(x1, y1, z1), new Punto(x2, y2, z2));
}
Pantalla *Pantalla::copia() {
Pantalla *c = new Pantalla();
Superficie *s = inicio;
while (s != 0) {
c->agregar(s->copia());
s = s->getSig(); // s++;
}
return c;
}
void Pantalla::girar(float ang) {
rotar(ejeVer, ang);
ejeHor->rotar(ejeVer, ang);
}
void Pantalla::mover(float ang) {
rotar(ejeHor, ang);
}
<file_sep>#ifndef VERTICE_H
#define VERTICE_H
#include "punto.h"
class Vertice : public Punto {
private:
Vertice *sig;
public:
Vertice(float, float, float);
Vertice *getSig();
void setSig(Vertice *);
Vertice *copia();
void desplegar();
};
#endif // VERTICE_H
<file_sep>#include "superficie.h"
#include "QtOpenGL"
Superficie::Superficie() {
inicio = 0;
final = 0;
sig = 0;
rojo = 0;
verde = 0;
azul = 0;
nx = 0;
ny = 0;
nz = 0;
}
Superficie::~Superficie() {
Vertice *aux = 0;
Vertice *v = inicio;
while (v != 0) {
aux = v->getSig();
delete v;
v = aux;
}
}
Superficie *Superficie::getSig() {
return sig;
}
void Superficie::setSig(Superficie *sig) {
this->sig = sig;
}
void Superficie::setColor(float rojo, float verde, float azul) {
this->rojo = rojo;
this->verde = verde;
this->azul = azul;
}
void Superficie::setNormal(float nx, float ny, float nz) {
this->nx = nx;
this->ny = ny;
this->nz = nz;
}
Superficie *Superficie::copia() {
Superficie *c = new Superficie();
Vertice *v = inicio;
while (v != 0) {
c->agregar(v->copia());
v = v->getSig(); // v++;
}
return c;
}
void Superficie::agregar(Vertice *v) {
if (inicio == 0) {
inicio = v;
final = v;
} else {
final->setSig(v);
final = v;
}
}
void Superficie::transformar(Matriz3D *m) {
Vertice *v = inicio;
while (v != 0) {
v->transformar(m);
v = v->getSig(); // v++;
}
}
void Superficie::desplegar() {
GLfloat materialDiffuse[] = { rojo, verde, azul, 1 };
glMaterialfv(GL_FRONT, GL_DIFFUSE, materialDiffuse);
glNormal3f(nx, ny, nz);
glBegin(GL_POLYGON);
Vertice *v = inicio;
while (v != 0) {
v->desplegar();
v = v->getSig(); // v++;
}
glEnd();
}
<file_sep>#ifndef MATRIZ3D_H
#define MATRIZ3D_H
class Matriz3D {
private:
float matriz[4][4];
public:
Matriz3D();
Matriz3D(float, float, float, float, float, float, float, float, float, float, float, float);
float getDato(int, int);
void setDato(int, int, float);
Matriz3D *copia();
Matriz3D *mult(Matriz3D *);
};
#endif // MATRIZ3D_H
<file_sep>#include "hombro.h"
Hombro::Hombro() : Objeto3D() {
}
Eje *Hombro::getEjeVer() {
return ejeVer;
}
Eje *Hombro::getEjeHor() {
return ejeHor;
}
void Hombro::setEjeVer(Eje *ejeVer) {
this->ejeVer = ejeVer;
}
void Hombro::setEjeVer(float x1, float y1, float z1, float x2, float y2, float z2) {
ejeVer = new Eje(new Punto(x1, y1, z1), new Punto(x2, y2, z2));
}
void Hombro::setEjeHor(Eje *ejeHor) {
this->ejeHor = ejeHor;
}
void Hombro::setEjeHor(float x1, float y1, float z1, float x2, float y2, float z2) {
ejeHor = new Eje(new Punto(x1, y1, z1), new Punto(x2, y2, z2));
}
Hombro *Hombro::copia() {
Hombro *c = new Hombro();
Superficie *s = inicio;
while (s != 0) {
c->agregar(s->copia());
s = s->getSig(); // s++;
}
return c;
}
void Hombro::girar(float ang) {
rotar(ejeVer, ang);
ejeHor->rotar(ejeVer, ang);
}
void Hombro::mover(float ang) {
rotar(ejeHor, ang);
}
<file_sep>#include "antebrazo.h"
Antebrazo::Antebrazo() : Objeto3D() {
}
Eje *Antebrazo::getEjeVer() {
return ejeVer;
}
Eje *Antebrazo::getEjeHor() {
return ejeHor;
}
void Antebrazo::setEjeVer(Eje *ejeVer) {
this->ejeVer = ejeVer;
}
void Antebrazo::setEjeVer(float x1, float y1, float z1, float x2, float y2, float z2) {
ejeVer = new Eje(new Punto(x1, y1, z1), new Punto(x2, y2, z2));
}
void Antebrazo::setEjeHor(Eje *ejeHor) {
this->ejeHor = ejeHor;
}
void Antebrazo::setEjeHor(float x1, float y1, float z1, float x2, float y2, float z2) {
ejeHor = new Eje(new Punto(x1, y1, z1), new Punto(x2, y2, z2));
}
Antebrazo *Antebrazo::copia() {
Antebrazo *c = new Antebrazo();
Superficie *s = inicio;
while (s != 0) {
c->agregar(s->copia());
s = s->getSig(); // s++;
}
return c;
}
void Antebrazo::girar(float ang) {
rotar(ejeVer, ang);
ejeHor->rotar(ejeVer, ang);
}
void Antebrazo::mover(float ang) {
rotar(ejeHor, ang);
}
<file_sep>#include "punto.h"
Punto::Punto() {
x = 0;
y = 0;
z = 0;
}
Punto::Punto(float x, float y, float z) {
this->x = x;
this->y = y;
this->z = z;
}
float Punto::getX() {
return x;
}
float Punto::getY() {
return y;
}
float Punto::getZ() {
return z;
}
void Punto::setX(float x) {
this->x = x;
}
void Punto::setY(float y) {
this->y = y;
}
void Punto::setZ(float z) {
this->z = z;
}
Punto *Punto::copia() {
return new Punto(x, y, z);
}
void Punto::transformar(Matriz3D *m)
{
float xa = x;
float ya = y;
float za = z;
float A = m->getDato(0, 0);
float B = m->getDato(0, 1);
float C = m->getDato(0, 2);
float D = m->getDato(0, 3);
float E = m->getDato(1, 0);
float F = m->getDato(1, 1);
float G = m->getDato(1, 2);
float H = m->getDato(1, 3);
float I = m->getDato(2, 0);
float J = m->getDato(2, 1);
float K = m->getDato(2, 2);
float L = m->getDato(2, 3);
x = (float) (A * xa + B * ya + C * za + D);
y = (float) (E * xa + F * ya + G * za + H);
z = (float) (I * xa + J * ya + K * za + L);
}
<file_sep>#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include <QKeyEvent>
#include "brazo.h"
extern int cx;
extern int cy;
extern int cz;
extern int estado;
extern int timer;
extern int tp;
extern Brazo *brazo;
namespace Ui {
class MainWindow;
}
class MainWindow : public QMainWindow {
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = 0);
~MainWindow();
void brazoMovP();
void brazoMovN();
private slots:
void on_actionAbrir_triggered();
void on_actionGirar_Hombro_triggered();
void on_actionMover_Hombro_triggered();
void on_actionMover_Antebrazo_triggered();
void on_actionGirar_Pantalla_triggered();
void on_actionMover_Pantalla_triggered();
private:
Ui::MainWindow *ui;
int timerId;
protected:
void timerEvent(QTimerEvent *);
void keyPressEvent(QKeyEvent *);
};
#endif // MAINWINDOW_H
<file_sep>#include "vertice.h"
#include "QtOpenGL"
Vertice::Vertice(float x, float y, float z) : Punto(x, y, z) {
sig = 0;
}
Vertice *Vertice::getSig() {
return sig;
}
void Vertice::setSig(Vertice *sig) {
this->sig = sig;
}
Vertice *Vertice::copia() {
Vertice *c = new Vertice(x, y, z);
c->setSig(sig);
return c;
}
void Vertice::desplegar() {
glVertex3f(x, y, z);
}
<file_sep>#ifndef LINEA_H
#define LINEA_H
#include "punto.h"
class Linea {
protected:
Punto *p1;
Punto *p2;
public:
Linea();
Linea(Punto *, Punto *);
Linea(float, float, float, float, float, float);
Punto *getP1();
Punto *getP2();
void setP1(Punto *);
void setP1(float, float, float);
void setP2(Punto *);
void setP2(float, float, float);
Linea *copia();
void transformar(Matriz3D *);
};
#endif // LINEA_H
<file_sep>#include "mainwindow.h"
#include "ui_mainwindow.h"
int cx = 0;
int cy = 0;
int cz = 18;
int estado = -1;
int timer = 0;
int tp = 0;
Brazo *brazo = 0;
MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent), ui(new Ui::MainWindow) {
ui->setupUi(this);
timerId = startTimer(100);
}
MainWindow::~MainWindow() {
killTimer(timerId);
delete ui;
}
void MainWindow::brazoMovP() {
switch (estado) {
case 0: {
// Girar Hombro.
brazo->girarHombro(0.01);
timer = 1;
break;
}
case 1: {
// Mover Hombro.
brazo->moverHombro(0.01);
timer = 1;
break;
}
case 2: {
// Mover Antebrazo.
brazo->moverAntebrazo(0.01);
timer = 1;
break;
}
case 3: {
// Girar Pantalla.
brazo->girarPantalla(0.01);
timer = 1;
break;
}
case 4: {
// Mover Pantalla.
brazo->moverPantalla(0.01);
timer = 1;
break;
}
}
}
void MainWindow::brazoMovN() {
switch (estado) {
case 0: {
// Girar Hombro.
brazo->girarHombro(-0.01);
timer = 1;
break;
}
case 1: {
// Mover Hombro.
brazo->moverHombro(-0.01);
timer = 1;
break;
}
case 2: {
// Mover Antebrazo.
brazo->moverAntebrazo(-0.01);
timer = 1;
break;
}
case 3: {
// Girar Pantalla.
brazo->girarPantalla(-0.01);
timer = 1;
break;
}
case 4: {
// Mover Pantalla.
brazo->moverPantalla(-0.01);
timer = 1;
break;
}
}
}
void MainWindow::timerEvent(QTimerEvent *) {
if (tp != 0) {
if (tp == 1) {
brazoMovP();
} else if (tp == 2) {
brazoMovN();
}
}
if (timer != 0) {
ui->widget_1->updateGL();
ui->widget_2->updateGL();
ui->widget_3->updateGL();
ui->widget_4->updateGL();
timer = 0;
}
}
void MainWindow::keyPressEvent(QKeyEvent *event) {
if (event->key() == Qt::Key_Right) {
cx++;
timer = 1;
} else if (event->key() == Qt::Key_Left) {
cx--;
timer = 1;
} else if (event->key() == Qt::Key_Up) {
cy++;
timer = 1;
} else if (event->key() == Qt::Key_Down) {
cy--;
timer = 1;
} else if (event->key() == Qt::Key_Q) {
cz++;
timer = 1;
} else if (event->key() == Qt::Key_E) {
cz--;
timer = 1;
} else if (event->key() == Qt::Key_Z) {
brazoMovP();
} else if (event->key() == Qt::Key_X) {
brazoMovN();
}
}
void MainWindow::on_actionAbrir_triggered() {
QString dir = QFileDialog::getOpenFileName(this, "Abrir");
QFile file(dir);
file.open(QIODevice::ReadOnly | QIODevice::Text);
QDomDocument document;
document.setContent(&file);
brazo = new Brazo();
brazo->abrir(document);
timer = 1;
}
void MainWindow::on_actionGirar_Hombro_triggered() {
estado = 0;
}
void MainWindow::on_actionMover_Hombro_triggered() {
estado = 1;
}
void MainWindow::on_actionMover_Antebrazo_triggered() {
estado = 2;
}
void MainWindow::on_actionGirar_Pantalla_triggered() {
estado = 3;
}
void MainWindow::on_actionMover_Pantalla_triggered() {
estado = 4;
}
<file_sep>#ifndef HOMBRO_H
#define HOMBRO_H
#include "objeto3d.h"
class Hombro : public Objeto3D {
private:
Eje *ejeVer;
Eje *ejeHor;
public:
Hombro();
Eje *getEjeVer();
Eje *getEjeHor();
void setEjeVer(Eje *);
void setEjeVer(float, float, float, float, float, float);
void setEjeHor(Eje *);
void setEjeHor(float, float, float, float, float, float);
Hombro *copia();
void girar(float);
void mover(float);
};
#endif // HOMBRO_H
<file_sep>#include "widget.h"
#include "mainwindow.h"
#include "QtOpenGL"
#include "GL/glu.h"
int cwidget = 0;
int xa = 0;
Widget::Widget(QWidget *parent) : QGLWidget(parent) {
}
void Widget::initializeGL() {
qglClearColor(Qt::white);
glEnable(GL_DEPTH_TEST);
glEnable(GL_LIGHTING);
glEnable(GL_LIGHT0);
glEnable(GL_LIGHT1);
GLfloat lightPosition[] = { 0, 20, 15, 1 };
glLightfv(GL_LIGHT0, GL_POSITION, lightPosition);
GLfloat lightAmbient[] = { 1, 1, 1, 0 };
glLightfv(GL_LIGHT1, GL_AMBIENT, lightAmbient);
}
void Widget::resizeGL(int w, int h) {
glViewport(0, 0, w, h);
}
void Widget::paintGL() {
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluPerspective(45, 1, 1, 100);
switch (cwidget) {
case 0: {
gluLookAt(cx, cy, cz, 0, 0, 0, 0, 1, 0);
cwidget++;
break;
}
case 1: {
// Lateral derecha.
gluLookAt(18, 5, 0, 0, 0, 0, 0, 1, 0);
cwidget++;
break;
}
case 2: {
// Vista superior.
gluLookAt(0, 18, 5, 0, 0, 0, 0, 0, -1);
cwidget++;
break;
}
case 3: {
// Vista posterior.
gluLookAt(0, 5, -18, 0, 0, 0, 0, 1, 0);
cwidget = 0;
break;
}
}
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
glTranslatef(-5, -3, 4);
if (brazo != 0) {
brazo->desplegar();
}
}
void Widget::mousePressEvent(QMouseEvent *event) {
if (event->button() == Qt::LeftButton) {
tp = 1;
} else if (event->button() == Qt::RightButton) {
tp = 2;
}
}
void Widget::mouseMoveEvent(QMouseEvent *event) {
float x = event->x();
switch (estado) {
case 0: {
// Girar Hombro.
if (x > xa) {
brazo->girarHombro(0.01);
} else {
brazo->girarHombro(-0.01);
}
xa = x;
timer = 1;
break;
}
case 1: {
// Mover Hombro.
if (x > xa) {
brazo->moverHombro(0.01);
} else {
brazo->moverHombro(-0.01);
}
xa = x;
timer = 1;
break;
}
case 2: {
// Mover Antebrazo.
if (x > xa) {
brazo->moverAntebrazo(0.01);
} else {
brazo->moverAntebrazo(-0.01);
}
xa = x;
timer = 1;
break;
}
case 3: {
// Girar Pantalla.
if (x > xa) {
brazo->girarPantalla(0.01);
} else {
brazo->girarPantalla(-0.01);
}
xa = x;
timer = 1;
break;
}
case 4: {
// Mover Pantalla.
if (x > xa) {
brazo->moverPantalla(0.01);
} else {
brazo->moverPantalla(-0.01);
}
xa = x;
timer = 1;
break;
}
}
}
void Widget::mouseReleaseEvent(QMouseEvent *) {
tp = 0;
}
<file_sep>#include "objeto3d.h"
#include "cmath"
Objeto3D::Objeto3D() {
inicio = 0;
final = 0;
}
Objeto3D::~Objeto3D() {
}
Superficie *Objeto3D::getInicio() {
return inicio;
}
Superficie *Objeto3D::getFinal() {
return final;
}
void Objeto3D::setInicio(Superficie *inicio) {
this->inicio = inicio;
}
void Objeto3D::setFinal(Superficie *final) {
this->final = final;
}
Objeto3D *Objeto3D::copia() {
Objeto3D *c = new Objeto3D();
Superficie *s = inicio;
while (s != 0) {
c->agregar(s->copia());
s = s->getSig(); // s++;
}
return c;
}
void Objeto3D::agregar(Superficie *s) {
if (inicio == 0) {
inicio = s;
final = s;
} else {
final->setSig(s);
final = s;
}
}
void Objeto3D::transformar(Matriz3D *m) {
Superficie *s = inicio;
while (s != 0) {
s->transformar(m);
s = s->getSig(); // s++;
}
}
void Objeto3D::rotar(Eje *eje, float ang) {
float x1 = eje->getP1()->getX();
float y1 = eje->getP1()->getY();
float z1 = eje->getP1()->getZ();
float x2 = eje->getP2()->getX();
float y2 = eje->getP2()->getY();
float z2 = eje->getP2()->getZ();
float a = x2 - x1;
float b = y2 - y1;
float c = z2 - z1;
float alfa = atan(b / c);
if (isnan(alfa)) {
alfa = 3.1416;
}
if (c < 0) {
alfa += 3.1416;
}
float d = sqrt(pow(b, 2) + pow(c, 2));
float beta = atan(a / d);
Matriz3D *T1 = new Matriz3D(
1, 0, 0, -x1,
0, 1, 0, -y1,
0, 0, 1, -z1); // -T
Matriz3D *Rx1 = new Matriz3D(
1, 0, 0, 0,
0, cos(alfa), -sin(alfa), 0,
0, sin(alfa), cos(alfa), 0); // +A
Matriz3D *Ry1 = new Matriz3D(
cos(-beta), 0, sin(-beta), 0,
0, 1, 0, 0,
-sin(-beta), 0, cos(-beta), 0); // -B
Matriz3D *Rz = new Matriz3D(
cos(ang), -sin(ang), 0, 0,
sin(ang), cos(ang), 0, 0,
0, 0, 1, 0);
Matriz3D *Ry2 = new Matriz3D(
cos(beta), 0, sin(beta), 0,
0, 1, 0, 0,
-sin(beta), 0, cos(beta), 0); // +B
Matriz3D *Rx2 = new Matriz3D(
1, 0, 0, 0,
0, cos(-alfa), -sin(-alfa), 0,
0, sin(-alfa), cos(-alfa), 0); // -A
Matriz3D *T2 = new Matriz3D(
1, 0, 0, x1,
0, 1, 0, y1,
0, 0, 1, z1); // +T
Matriz3D *M = T2->mult(Rx2->mult(Ry2->mult(Rz->mult(Ry1->mult(Rx1->mult(T1))))));
transformar(M);
}
void Objeto3D::desplegar() {
Superficie *s = inicio;
while (s != 0) {
s->desplegar();
s = s->getSig(); // s++;
}
}
<file_sep>#ifndef BRAZO_H
#define BRAZO_H
#include "QtXml"
#include "qfiledialog.h"
#include "hombro.h"
#include "antebrazo.h"
#include "pantalla.h"
class Brazo {
private:
Hombro *hombro;
Antebrazo *antebrazo;
Pantalla *pantalla;
public:
Brazo();
Hombro *getHombro();
Antebrazo *getAntebrazo();
Pantalla *getPantalla();
void setHombro(Hombro *);
void setAntebrazo(Antebrazo *);
void setPantalla(Pantalla *);
Brazo *copia();
void abrir(QDomDocument);
void girarHombro(float);
void moverHombro(float);
void moverAntebrazo(float);
void girarPantalla(float);
void moverPantalla(float);
void desplegar();
};
#endif // BRAZO_H
<file_sep>#include "eje.h"
#include "cmath"
#include "QtOpenGL"
Eje::Eje(Punto *p1, Punto *p2) : Linea(p1, p2) {
}
void Eje::rotar(Eje *eje, float ang) {
float x1 = eje->getP1()->getX();
float y1 = eje->getP1()->getY();
float z1 = eje->getP1()->getZ();
float x2 = eje->getP2()->getX();
float y2 = eje->getP2()->getY();
float z2 = eje->getP2()->getZ();
float a = x2 - x1;
float b = y2 - y1;
float c = z2 - z1;
float alfa = atan(b / c);
if (isnan(alfa)) {
alfa = 3.1416;
}
if (c < 0) {
alfa += 3.1416;
}
float d = sqrt(pow(b, 2) + pow(c, 2));
float beta = atan(a / d);
Matriz3D *T1 = new Matriz3D(
1, 0, 0, -x1,
0, 1, 0, -y1,
0, 0, 1, -z1); // -T
Matriz3D *Rx1 = new Matriz3D(
1, 0, 0, 0,
0, cos(alfa), -sin(alfa), 0,
0, sin(alfa), cos(alfa), 0); // +A
Matriz3D *Ry1 = new Matriz3D(
cos(-beta), 0, sin(-beta), 0,
0, 1, 0, 0,
-sin(-beta), 0, cos(-beta), 0); // -B
Matriz3D *Rz = new Matriz3D(
cos(ang), -sin(ang), 0, 0,
sin(ang), cos(ang), 0, 0,
0, 0, 1, 0);
Matriz3D *Ry2 = new Matriz3D(
cos(beta), 0, sin(beta), 0,
0, 1, 0, 0,
-sin(beta), 0, cos(beta), 0); // +B
Matriz3D *Rx2 = new Matriz3D(
1, 0, 0, 0,
0, cos(-alfa), -sin(-alfa), 0,
0, sin(-alfa), cos(-alfa), 0); // -A
Matriz3D *T2 = new Matriz3D(
1, 0, 0, x1,
0, 1, 0, y1,
0, 0, 1, z1); // +T
Matriz3D *M = T2->mult(Rx2->mult(Ry2->mult(Rz->mult(Ry1->mult(Rx1->mult(T1))))));
transformar(M);
}
void Eje::desplegar() {
glLineWidth(1);
glColor3f(1, 0, 0);
glBegin(GL_LINES);
glVertex3f(p1->getX(), p1->getY(), p1->getZ());
glVertex3f(p2->getX(), p2->getY(), p2->getZ());
glEnd();
}
<file_sep>#include "brazo.h"
Brazo::Brazo() {
hombro = new Hombro();
antebrazo = new Antebrazo();
pantalla = new Pantalla();
}
Hombro *Brazo::getHombro() {
return hombro;
}
Antebrazo *Brazo::getAntebrazo() {
return antebrazo;
}
Pantalla *Brazo::getPantalla() {
return pantalla;
}
void Brazo::setHombro(Hombro *hombro) {
this->hombro = hombro;
}
void Brazo::setAntebrazo(Antebrazo *antebrazo) {
this->antebrazo = antebrazo;
}
void Brazo::setPantalla(Pantalla *pantalla) {
this->pantalla = pantalla;
}
Brazo *Brazo::copia() {
Brazo *c = new Brazo();
c->setHombro(hombro->copia());
c->setAntebrazo(antebrazo->copia());
c->setPantalla(pantalla->copia());
return c;
}
void Brazo::abrir(QDomDocument qd) {
QDomElement brazo = qd.firstChildElement();
QDomElement objeto = brazo.firstChildElement();
while (objeto.isElement()) {
// Vectores verticales y horizontales.
float vx1 = objeto.attribute("VX1").toFloat();
float vy1 = objeto.attribute("VY1").toFloat();
float vz1 = objeto.attribute("VZ1").toFloat();
float vx2 = objeto.attribute("VX2").toFloat();
float vy2 = objeto.attribute("VY2").toFloat();
float vz2 = objeto.attribute("VZ2").toFloat();
float hx1 = objeto.attribute("HX1").toFloat();
float hy1 = objeto.attribute("HY1").toFloat();
float hz1 = objeto.attribute("HZ1").toFloat();
float hx2 = objeto.attribute("HX2").toFloat();
float hy2 = objeto.attribute("HY2").toFloat();
float hz2 = objeto.attribute("HZ2").toFloat();
if (objeto.tagName() == "Hombro") {
hombro->setEjeVer(vx1, vy1, vz1, vx2, vy2, vz2);
hombro->setEjeHor(hx1, hy1, hz1, hx2, hy2, hz2);
} else if (objeto.tagName() == "Antebrazo") {
antebrazo->setEjeVer(vx1, vy1, vz1, vx2, vy2, vz2);
antebrazo->setEjeHor(hx1, hy1, hz1, hx2, hy2, hz2);
} else if (objeto.tagName() == "Pantalla") {
pantalla->setEjeVer(vx1, vy1, vz1, vx2, vy2, vz2);
pantalla->setEjeHor(hx1, hy1, hz1, hx2, hy2, hz2);
}
QDomElement superficie = objeto.firstChildElement();
while (superficie.isElement()) {
float rojo = superficie.attribute("ROJO").toFloat();
float verde = superficie.attribute("VERDE").toFloat();
float azul = superficie.attribute("AZUL").toFloat();
float nx = superficie.attribute("NX").toFloat();
float ny = superficie.attribute("NY").toFloat();
float nz = superficie.attribute("NZ").toFloat();
Superficie *s = new Superficie();
s->setColor(rojo, verde, azul);
s->setNormal(nx, ny, nz);
QDomElement vertice = superficie.firstChildElement();
while (vertice.isElement()) {
float x = vertice.attribute("X").toFloat();
float y = vertice.attribute("Y").toFloat();
float z = vertice.attribute("Z").toFloat();
Vertice *v = new Vertice(x, y, z);
s->agregar(v);
vertice = vertice.nextSiblingElement(); // v++;
}
if (objeto.tagName() == "Hombro") {
hombro->agregar(s);
} else if (objeto.tagName() == "Antebrazo") {
antebrazo->agregar(s);
} else if (objeto.tagName() == "Pantalla") {
pantalla->agregar(s);
}
superficie = superficie.nextSiblingElement(); // s++;
}
objeto = objeto.nextSiblingElement(); // o++;
}
}
void Brazo::girarHombro(float ang) {
hombro->girar(ang);
antebrazo->rotar(hombro->getEjeVer(), ang);
antebrazo->getEjeVer()->rotar(hombro->getEjeVer(), ang);
antebrazo->getEjeHor()->rotar(hombro->getEjeVer(), ang);
pantalla->rotar(hombro->getEjeVer(), ang);
pantalla->getEjeVer()->rotar(hombro->getEjeVer(), ang);
pantalla->getEjeHor()->rotar(hombro->getEjeVer(), ang);
}
void Brazo::moverHombro(float ang) {
hombro->mover(ang);
antebrazo->rotar(hombro->getEjeHor(), ang);
antebrazo->getEjeVer()->rotar(hombro->getEjeHor(), ang);
antebrazo->getEjeHor()->rotar(hombro->getEjeHor(), ang);
pantalla->rotar(hombro->getEjeHor(), ang);
pantalla->getEjeVer()->rotar(hombro->getEjeHor(), ang);
pantalla->getEjeHor()->rotar(hombro->getEjeHor(), ang);
}
void Brazo::moverAntebrazo(float ang) {
antebrazo->mover(ang);
pantalla->rotar(antebrazo->getEjeHor(), ang);
pantalla->getEjeVer()->rotar(antebrazo->getEjeHor(), ang);
pantalla->getEjeHor()->rotar(antebrazo->getEjeHor(), ang);
}
void Brazo::girarPantalla(float ang) {
pantalla->girar(ang);
}
void Brazo::moverPantalla(float ang) {
pantalla->mover(ang);
}
void Brazo::desplegar() {
hombro->desplegar();
hombro->getEjeVer()->desplegar();
hombro->getEjeHor()->desplegar();
antebrazo->desplegar();
antebrazo->getEjeVer()->desplegar();
antebrazo->getEjeHor()->desplegar();
pantalla->desplegar();
pantalla->getEjeVer()->desplegar();
pantalla->getEjeHor()->desplegar();
}
<file_sep>#ifndef OBJETO3D_H
#define OBJETO3D_H
#include "superficie.h"
#include "eje.h"
class Objeto3D {
protected:
Superficie *inicio;
Superficie *final;
public:
Objeto3D();
~Objeto3D();
Superficie *getInicio();
Superficie *getFinal();
void setInicio(Superficie *);
void setFinal(Superficie *);
Objeto3D *copia();
void agregar(Superficie *);
void transformar(Matriz3D *);
void rotar(Eje *, float);
void desplegar();
};
#endif // OBJETO3D_H
<file_sep>#ifndef EJE_H
#define EJE_H
#include "linea.h"
class Eje : public Linea {
public:
Eje(Punto *, Punto *);
void rotar(Eje *, float);
void desplegar();
};
#endif // EJE_H
<file_sep>#include "linea.h"
Linea::Linea() {
p1 = new Punto();
p2 = new Punto();
}
Linea::Linea(Punto *p1, Punto *p2) {
this->p1 = p1;
this->p2 = p2;
}
Linea::Linea(float x1, float y1, float z1, float x2, float y2, float z2) {
p1 = new Punto(x1, y1, z1);
p2 = new Punto(x2, y2, z2);
}
Punto *Linea::getP1() {
return p1;
}
Punto *Linea::getP2() {
return p2;
}
void Linea::setP1(Punto *p1) {
this->p1 = p1;
}
void Linea::setP1(float x, float y, float z) {
p1 = new Punto(x, y, z);
}
void Linea::setP2(Punto *p2) {
this->p2 = p2;
}
void Linea::setP2(float x, float y, float z) {
p2 = new Punto(x, y, z);
}
Linea *Linea::copia() {
return new Linea(p1->copia(), p2->copia());
}
void Linea::transformar(Matriz3D *m) {
p1->transformar(m);
p2->transformar(m);
}
<file_sep>#ifndef WIDGET_H
#define WIDGET_H
#include <QGLWidget>
class Widget : public QGLWidget {
Q_OBJECT
public:
explicit Widget(QWidget *parent = 0);
public slots:
void initializeGL();
void resizeGL(int, int);
void paintGL();
void mousePressEvent(QMouseEvent *);
void mouseMoveEvent(QMouseEvent *);
void mouseReleaseEvent(QMouseEvent *);
};
#endif // WIDGET_H
<file_sep>#include "matriz3d.h"
Matriz3D::Matriz3D() {
matriz[0][0] = 1;
matriz[0][1] = 0;
matriz[0][2] = 0;
matriz[0][3] = 0;
matriz[1][0] = 0;
matriz[1][1] = 1;
matriz[1][2] = 0;
matriz[1][3] = 0;
matriz[2][0] = 0;
matriz[2][1] = 0;
matriz[2][2] = 1;
matriz[2][0] = 0;
matriz[3][0] = 0;
matriz[3][1] = 0;
matriz[3][2] = 0;
matriz[3][3] = 1;
}
Matriz3D::Matriz3D(float a, float b, float c, float d,
float e, float f, float g, float h,
float i, float j, float k, float l) {
matriz[0][0] = a;
matriz[0][1] = b;
matriz[0][2] = c;
matriz[0][3] = d;
matriz[1][0] = e;
matriz[1][1] = f;
matriz[1][2] = g;
matriz[1][3] = h;
matriz[2][0] = i;
matriz[2][1] = j;
matriz[2][2] = k;
matriz[2][3] = l;
matriz[3][0] = 0;
matriz[3][1] = 0;
matriz[3][2] = 0;
matriz[3][3] = 1;
}
float Matriz3D::getDato(int i, int j) {
return matriz[i][j];
}
void Matriz3D::setDato(int i, int j, float d) {
matriz[i][j] = d;
}
Matriz3D *Matriz3D::copia() {
Matriz3D *c = new Matriz3D();
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
c->setDato(i, j, matriz[i][j]);
}
}
return c;
}
Matriz3D *Matriz3D::mult(Matriz3D *obj) {
Matriz3D *aux = new Matriz3D();
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
float d =
matriz[i][0] * obj->getDato(0, j) +
matriz[i][1] * obj->getDato(1, j) +
matriz[i][2] * obj->getDato(2, j) +
matriz[i][3] * obj->getDato(3, j);
aux->setDato(i, j, d);
}
}
return aux;
}
| 06321a0771c19ab24a717a24bc7495833dc08f57 | [
"C++"
] | 23 | C++ | raky291/QtOpenGL | c05d390627f09335dfe48cae1da3ca518753d9a3 | de7f19b1cac6c0456b567f446a0f22421bbb1634 | |
refs/heads/master | <file_sep>library(dplyr)
library(DT)
library(shiny)
library(googleVis)
library(ggplot2)
library(leaflet)
library(maps)
library(scales)
library(gtable)
library(ggpubr)
########## REORDER LEVELS ###########
df$sponsor_type <-factor(df$sponsor_type,
levels = c("US Fed", "NIH", "Industry", "Other"))
df$condition_type <-factor(df$condition_type,
levels = c("Type 1", "Type 2", "Diabetes", "Other"))
df$status_new <-factor(df$status_new,
levels = c("Not yet recruiting", "Recruiting", "Active, not recruiting", "Completed", "Other"))
df$enrollment_n <-factor(df$enrollment_n,
levels = c("<=50", "50-100", "100-1,000", "1,000-5,000", "5,000-10,000", ">10,000"))
df$phase <-factor(df$phase,
levels = c("Early Phase 1", "Phase 1", "Phase 1/Phase 2", "Phase 2",
"Phase 2/Phase 3", "Phase 3", "Phase 4", "N/A"))
df$duration_n <-factor(df$duration_n,
levels = c("<= 1 year", "1-2 years", "2-5 years", "5-10 years", "10+ years"))
########## LAST-MINUTE FILTERS ###########
#study type
df_interventional = df %>% filter(., study_type == "Clinical Trial")
df_interventional_not_NA = df_interventional %>% filter(., intervention_type != 'NA')
df_observational = df %>% filter(., study_type == "Observational Study")
df_intervention_type_not_NA = df %>% filter(., intervention_type != 'NA')
#sponsors
df1 = df %>% filter(., sponsor_type == "US Fed")
df2 = df %>% filter(., sponsor_type == "NIH")
df3 = df %>% filter(., sponsor_type == "Industry")
df4 = df %>% filter(., sponsor_type == "Other")
df_enrollment_not_NA = df %>% filter(., enrollment_n != 'NA')
df_duration_not_NA = df %>% filter(., duration_n != 'NA')
#data table
df_by_study = df %>% group_by(., study_type) %>% summarise(., n())
names(df_by_study) = c("study_type", "n")
################################
shinyServer(function(input, output){
### STUDY TYPES ###
output$studyinfo <- renderPlot(
if (input$chosen == "Sponsor Type") {
num_trials = df %>%
group_by(., study_type, sponsor_type) %>%
summarise(., num_trials = n())
df_sponsor = inner_join(df, num_trials, c("study_type","sponsor_type"))
g <- ggplot(data = df_sponsor, aes(x = sponsor_type))
g + geom_bar(aes(fill = sponsor_type)) +
geom_text(stat = 'count', aes(label = num_trials), size=3.5, vjust=-.5) +
labs(
#title = "Sponsor Type by Study Type",
x = "Sponsor Type by Study Type",
y = "Number of Studies",
fill = "Sponsor Type") +
theme(
#plot.title = element_text(color="black", size=20, face="bold.italic"),
axis.text = element_text(size = 13),
axis.title.x = element_text(color="black", size=16),# face="bold"),
axis.title.y = element_text(color="black", size=16),# face="bold"),
strip.text.x = element_text(size=16, color="black") #, face="bold")
# panel.background = element_rect(fill = "lightgrey",
# colour = "lightblue",
# size = 2.0, linetype = "solid"),
# panel.grid.major = element_line(size = 0.5, linetype = 'solid',
# colour = "white"),
# panel.grid.minor = element_line(size = 0.25, linetype = 'solid',
# colour = "white")
) +
facet_wrap( ~ study_type)
} else if (input$chosen == "Condition Type") {
num_trials = df %>%
group_by(., study_type, condition_type) %>%
summarise(., num_trials = n())
df_condition = inner_join(df, num_trials, c("study_type","condition_type"))
g <- ggplot(data = df_condition, aes(x = condition_type))
g + geom_bar(aes(fill = condition_type)) +
#geom_text(stat = 'count', aes(label = num_trials), size=3.5, vjust=-.5) +
geom_text(stat = 'count', aes(label = num_trials), size=3.5, vjust=-.5) +
labs(
#title = "Sponsor Type by Study Type",
x = "Condition Type by Study Type",
y = "Number of Studies",
fill = "Condition Type") +
theme(
#plot.title = element_text(color="black", size=20, face="bold.italic"),
axis.text = element_text(size = 13),
axis.title.x = element_text(color="black", size=16),
axis.title.y = element_text(color="black", size=16),
strip.text.x = element_text(size=16, color="black")
) +
facet_wrap( ~ study_type)
} else if (input$chosen == "Intervention Type") {
num_trials = df_intervention_type_not_NA %>%
group_by(., study_type, intervention_type) %>%
summarise(., num_trials = n())
df_intervention = inner_join(df, num_trials, c("study_type","intervention_type"))
g <- ggplot(data = df_intervention, aes(x = intervention_type))
g + geom_bar(aes(fill = intervention_type)) +
geom_text(stat = 'count', aes(label = num_trials), size=3.5, vjust=-.5) +
labs(
#title = "Sponsor Type by Study Type",
x = "Intervention Type by Study Type",
y = "Number of Studies",
fill = "Intervention Type") +
theme(
#plot.title = element_text(color="black", size=20, face="bold.italic"),
axis.text = element_text(size = 13),
axis.text.x = element_text(angle = 45, hjust = 1),
axis.title.x = element_text(color="black", size=16),
axis.title.y = element_text(color="black", size=16),
strip.text.x = element_text(size=16, color="black")
) +
facet_wrap( ~ study_type)
} else if (input$chosen == "Status") {
num_trials = df %>%
group_by(., study_type, status_new) %>%
summarise(., num_trials = n())
df_status = inner_join(df, num_trials, c("study_type","status_new"))
g <- ggplot(data = df_status, aes(x = status_new))
g + geom_bar(aes(fill = status_new)) +
geom_text(stat = 'count', aes(label = num_trials), size=3.5, vjust=-.5) +
labs(
#title = "Sponsor Type by Study Type",
x = "Status by Study Type",
y = "Number of Studies",
fill = "Status") +
theme(
#plot.title = element_text(color="black", size=20, face="bold.italic"),
axis.text = element_text(size = 13),
axis.text.x = element_text(angle = 45, hjust = 1),
axis.title.x = element_text(color="black", size=16),
axis.title.y = element_text(color="black", size=16),
strip.text.x = element_text(size=16, color="black")
) +
facet_wrap( ~ study_type)
} else if (input$chosen == "Enrollment") {
num_trials = df_enrollment_not_NA %>%
group_by(., study_type, enrollment_n) %>%
summarise(., num_trials = n())
df_enrollment = inner_join(df, num_trials, c("study_type","enrollment_n"))
g <- ggplot(data = df_enrollment, aes(x = enrollment_n))
g + geom_bar(aes(fill = enrollment_n)) +
geom_text(stat = 'count', aes(label = num_trials), size=3.5, vjust=-.5) +
labs(
#title = "Sponsor Type by Study Type",
x = "Enrollment by Study Type",
y = "Number of Studies",
fill = "Enrollment") +
theme(
#plot.title = element_text(color="black", size=20, face="bold.italic"),
axis.text = element_text(size = 13),
axis.text.x = element_text(angle = 45, hjust = 1),
axis.title.x = element_text(color="black", size=16),
axis.title.y = element_text(color="black", size=16),
strip.text.x = element_text(size=16, color="black")
) +
facet_wrap( ~ study_type)
} else if (input$chosen == "Phase (Clinical Trial)") {
num_trials = df_interventional_not_NA %>%
group_by(., study_type, phase) %>%
summarise(., num_trials = n())
df_phase = inner_join(df, num_trials, c("study_type","phase"))
g <- ggplot(data = df_phase, aes(x = phase))
g + geom_bar(aes(fill = phase)) +
geom_text(stat = 'count', aes(label = num_trials), size=3.5, vjust=-.5) +
labs(
#title = "Sponsor Type by Study Type",
x = "Phase (Clinical Trial)",
y = "Number of Studies",
fill = "Phase") +
theme(
#plot.title = element_text(color="black", size=20, face="bold.italic"),
axis.text = element_text(size = 13),
#axis.text.x = element_text(angle = 45, hjust = 1),
axis.title.x = element_text(color="black", size=16),
axis.title.y = element_text(color="black", size=16),
strip.text.x = element_text(size=16, color="black")
) +
facet_wrap( ~ study_type)
} else {
num_trials = df_duration_not_NA %>%
group_by(., study_type, duration_n) %>%
summarise(., num_trials = n())
df_duration = inner_join(df, num_trials, c("study_type","duration_n"))
g <- ggplot(data = df_duration, aes(x = duration_n))
g + geom_bar(aes(fill = duration_n)) +
geom_text(stat = 'count', aes(label = num_trials), size=3.5, vjust=-.5) +
labs(
#title = "Sponsor Type by Study Type",
x = "Duration by Study Type",
y = "Number of Studies",
fill = "Duration") +
theme(
#plot.title = element_text(color="black", size=20, face="bold.italic"),
axis.text = element_text(size = 13),
#axis.text.x = element_text(angle = 45, hjust = 1),
axis.title.x = element_text(color="black", size=16),
axis.title.y = element_text(color="black", size=16),
strip.text.x = element_text(size=16, color="black")
) +
facet_wrap( ~ study_type)
}, height = 650)
### TIME ###
output$time <- renderPlot({
if (input$sel == "Studies by Start Year") {
#sponsor
num_trials_sponsor = df %>% filter(., start_date_yr > 1990 & completion_date_yr < 2030) %>%
group_by(., start_date_yr, sponsor_type) %>%
summarise(., num_trials = n())
p1 <- ggplot(data=num_trials_sponsor, aes(x=start_date_yr, y=num_trials, group=sponsor_type)) +
geom_line(aes(linetype = sponsor_type, color = sponsor_type)) +
scale_linetype_manual(values=rep("solid", 4)) +
geom_point() +
labs(
title = "Number of Studies by Start Year and by Sponsor Type",
x = "Start Year",
y = "Number of Studies",
group = "Sponsor Type") +
theme(
plot.title = element_text(color="black", size=16, face="bold"),
axis.text = element_text(size = 13),
axis.title.x = element_text(color="black", size=16),
axis.title.y = element_text(color="black", size=16),
legend.position = c(0.15, 0.6)
)
#condition
num_trials_intervention = df_intervention_type_not_NA %>% filter(., start_date_yr > 1990 & completion_date_yr < 2030) %>%
group_by(., start_date_yr, intervention_type) %>%
summarise(., num_trials = n())
p2 <- ggplot(data=num_trials_intervention, aes(x=start_date_yr, y=num_trials, group=intervention_type)) +
geom_line(aes(linetype = intervention_type, color = intervention_type)) +
scale_linetype_manual(values=rep("solid", 7)) +
geom_point() +
labs(
title = "Number of Studies by Start Year and by Intervention Type",
x = "Start Year",
y = "Number of Studies",
group = "Intervention Type") +
theme(
plot.title = element_text(color="black", size=16, face="bold"),
axis.text = element_text(size = 13),
axis.title.x = element_text(color="black", size=16),
axis.title.y = element_text(color="black", size=16),
legend.position = c(0.15, 0.6)
)
ggarrange(p1, p2, ncol = 2, nrow = 1)
} else {
#sponsor
num_trials_sponsor = df %>% filter(., start_date_yr > 1990 & completion_date_yr < 2030) %>%
group_by(., completion_date_yr, sponsor_type) %>%
summarise(., num_trials = n())
p1 <- ggplot(data=num_trials_sponsor, aes(x=completion_date_yr, y=num_trials, group=sponsor_type)) +
geom_line(aes(linetype = sponsor_type, color = sponsor_type)) +
scale_linetype_manual(values=rep("solid", 4)) +
geom_point() +
labs(
title = "Number of Studies by Completion Year and by Sponsor Type",
x = "Completion Year",
y = "Number of Studies",
group = "Sponsor Type") +
theme(
plot.title = element_text(color="black", size=16, face="bold"),
axis.text = element_text(size = 13),
axis.title.x = element_text(color="black", size=16),
axis.title.y = element_text(color="black", size=16),
legend.position = c(0.85, 0.5)
)
#condition
num_trials_intervention = df_intervention_type_not_NA %>% filter(., start_date_yr > 1990 & completion_date_yr < 2030) %>%
group_by(., completion_date_yr, intervention_type) %>%
summarise(., num_trials = n())
p2 <- ggplot(data=num_trials_intervention, aes(x=completion_date_yr, y=num_trials, group=intervention_type)) +
geom_line(aes(linetype = intervention_type, color = intervention_type)) +
scale_linetype_manual(values=rep("solid", 7)) +
geom_point() +
labs(
title = "Number of Studies by Completion Year and by Intervention Type",
x = "Completion Year",
y = "Number of Studies",
group = "Intervention Type") +
theme(
plot.title = element_text(color="black", size=16, face="bold"),
axis.text = element_text(size = 13),
axis.title.x = element_text(color="black", size=16),
axis.title.y = element_text(color="black", size=16),
legend.position = c(0.85, 0.5)
)
ggarrange(p1, p2, ncol = 2, nrow = 1)
}
}, height = 650)
### SPONSOR ###
# output$sponsor <- renderPlot(
#
# if (input$selected == "sponsor_type") {
# ggplot(data = df, aes(x = sponsor_type)) + geom_bar(aes(fill = study_type), position = "dodge")
# } else if (input$selected == "sponsor") {
# ggplot(data = df, aes(x = sponsor_type)) + geom_bar(aes(fill = study_type), position = "dodge")
# } else {
# ggplot(data = df, aes(x = sponsor_num)) + geom_bar(aes(fill = study_type), position = "dodge")
# }
#
# )
# show data using DataTable
output$table <- DT::renderDataTable({
datatable(df_sponsor) #%>%
#formatStyle(input$selected, background="skyblue", fontWeight='bold')
})
### MAPS ###
output$mymap <- renderLeaflet({
if (input$choice == "All") {
leaflet() %>%
addTiles() %>%
addMarkers(data = df %>% filter(., study_type == "Clinical Trial"), group = "Clinical Trial", clusterOptions = markerClusterOptions()) %>%
addMarkers(data = df %>% filter(., study_type == "Observational Study"), group = "Observational Study", clusterOptions = markerClusterOptions()) %>%
addLayersControl(
baseGroups = c("Clinical Trial", "Observational Study"),
options = layersControlOptions(collapsed = FALSE),
position = c("topleft")
)
}
else if (input$choice == "US Fed") {
leaflet() %>%
addTiles() %>%
addMarkers(data = df1 %>% filter(., study_type == "Clinical Trial"), group = "Clinical Trial", clusterOptions = markerClusterOptions()) %>%
addMarkers(data = df1 %>% filter(., study_type == "Observational Study"), group = "Observational Study", clusterOptions = markerClusterOptions()) %>%
addLayersControl(
baseGroups = c("Clinical Trial", "Observational Study"),
options = layersControlOptions(collapsed = FALSE),
position = c("topleft")
)
} else if (input$choice == "NIH") {
leaflet() %>%
addTiles() %>%
addMarkers(data = df2 %>% filter(., study_type == "Clinical Trial"), group = "Clinical Trial", clusterOptions = markerClusterOptions()) %>%
addMarkers(data = df2 %>% filter(., study_type == "Observational Study"), group = "Observational Study", clusterOptions = markerClusterOptions()) %>%
addLayersControl(
baseGroups = c("Clinical Trial", "Observational Study"),
options = layersControlOptions(collapsed = FALSE),
position = c("topleft")
)
} else if (input$choice == "Industry") {
leaflet() %>%
addTiles() %>%
addMarkers(data = df3 %>% filter(., study_type == "Clinical Trial"), group = "Clinical Trial", clusterOptions = markerClusterOptions()) %>%
addMarkers(data = df3 %>% filter(., study_type == "Observational Study"), group = "Observational Study", clusterOptions = markerClusterOptions()) %>%
addLayersControl(
baseGroups = c("Clinical Trial", "Observational Study"),
options = layersControlOptions(collapsed = FALSE),
position = c("topleft")
)
} else {
leaflet() %>%
addTiles() %>%
addMarkers(data = df4 %>% filter(., study_type == "Clinical Trial"), group = "Clinical Trial", clusterOptions = markerClusterOptions()) %>%
addMarkers(data = df4 %>% filter(., study_type == "Observational Study"), group = "Observational Study", clusterOptions = markerClusterOptions()) %>%
addLayersControl(
baseGroups = c("Clinical Trial", "Observational Study"),
options = layersControlOptions(collapsed = FALSE),
position = c("topleft")
)
}
})
})
<file_sep># diabetes-app
This app is a prototype and was created mainly for educational purposes. It is a required project at the New York Data Science Academy Bootcamp (Cohort 13) and was completed in a span of approximately two weeks. The data was a subset of the diabetes studies in https://clinicaltrials.gov/ct2/home.
The actual app can be found at:
https://johnyap.shinyapps.io/clinicalstudiesApp/
For details about how to use the app and some insights about the data summaries and results, see my blog post here:
https://nycdatascience.com/blog/student-works/r-shiny/visualizing-diabetes-clinical-studies-data/
### additional notes:
In the Studies Info feature,
- In Condition Type, the category, Diabetes, includes studies that did not distinguish between the type of diabetes (Type 1 or 2). These may likely be Type 2 diabes because it is the most common type. The Other category includes studies of other less common diabetes types such as gestational diabetes or studies that do not specifically study diabetes but other diseases on diabetes patients.
- In Phase (Clinical Trial), the phase category N/A (or not applicable) represents trials without FDA-defined phases, including trials of devices or behavioral interventions.
### references:
http://www.cnn.com/interactive/2014/10/health/epidemics-through-history/
https://clinicaltrials.gov/ct2/home
<file_sep>library(DT)
library(shiny)
library(shinydashboard)
library(ggplot2)
library(leaflet)
library(dplyr)
shinyUI(dashboardPage(
dashboardHeader(title = "Diabetes Studies App"),
dashboardSidebar(
sidebarMenu(
menuItem("Intro", tabName = "intro", icon = icon("flag")),
menuItem("Studies Info", tabName = "studyinfo", icon = icon("ambulance")),
menuItem("Annual Data", tabName = "time", icon = icon("calendar")),
menuItem("Sponsor Data", tabName = "data", icon = icon("cog")),
menuItem("Map", tabName = "map", icon = icon("map"))
)
), #close dashboardSidebar
dashboardBody(
tags$head(
tags$link(rel = "stylesheet", type = "text/css", href = "custom.css")
),
tabItems(
tabItem(tabName = "intro",
fluidRow(
box(tags$iframe(src="https://www.youtube.com/embed/wmOW091P2ew", width="100%", height="400px")),
box(tags$iframe(src="https://www.youtube.com/embed/7Eg14Yp0TMY", width="100%", height="400px"))
)
),
tabItem(tabName = "studyinfo",
selectizeInput("chosen",
"Select category",
choice_study_info),
fluidRow(plotOutput("studyinfo"))
),
tabItem(tabName = "map",
fluidRow(box(leafletOutput("mymap", height=700), width = 10),
box(
selectizeInput("choice",
"Select category",
choice_map), width = 2
)
)
),
tabItem(tabName = "time",
selectInput(inputId = "sel",
label = 'Select category',
choices = choice_time,
selected = choice_time), #select shows at the top
fluidRow(plotOutput("time"))
),
tabItem(tabName = "data",
fluidRow(box(DT::dataTableOutput("table"),
width = 12))
)
) #close tabItems
) #close dashboardBody
) #close dashboardPage
) #close shinyUI
<file_sep>## global.R ##
### READ DATASET
library(dplyr)
df = read.csv("./data/diabetes_n.csv")
df = df %>%
mutate(., study_type = ifelse(study_type == "Interventional", "Clinical Trial", "Observational Study"))
### SIDEBAR MENU ITEMS
choice_map = c("All","US Fed", "NIH", "Industry", "Other")
choice_study_info = c("Sponsor Type", "Condition Type", "Intervention Type", "Status", "Enrollment", "Phase (Clinical Trial)", "Duration")
choice_sponsor = c("sponsor_type", "sponsor", "sponsor_num")
choice_time = c("Studies by Start Year", "Studies by Completion Year")
#### SPONSOR TABLE
# duration
df_duration = df %>%
filter(., !is.na(duration)) %>%
group_by(., sponsor) %>%
summarise(.,
duration.mean = round(mean(duration), 1),
duration.min = round(min(duration), 1),
duration.max = round(max(duration), 1))
df_enrollment = df %>%
filter(., !is.na(enrollment)) %>%
group_by(., sponsor) %>%
summarise(.,
enrollment.total = sum(enrollment),
enrollment.mean = round(mean(enrollment), 0),
enrollment.min = min(enrollment),
enrollment.max = max(enrollment))
df_num_studies = df %>%
group_by(., sponsor) %>%
summarise(., studies.total = n())
df_1 = inner_join(df_num_studies, df_enrollment, by = "sponsor")
df_sponsor = inner_join(df_1, df_duration, by = "sponsor")
| 3ae27ae7ed692308fed378ca26f8dec9166be8d6 | [
"Markdown",
"R"
] | 4 | R | DrJohan/diabetes-app | 8dd1b6507c042b9ef20993671939c0bc936b6d1c | f1ba83c7f340bead94d3ef4edb8fbfb1049f5860 | |
refs/heads/master | <repo_name>rubycoder/glimmer-cs-timer<file_sep>/CHANGELOG.md
# Change Log
## 1.2.0
- Upgrade to support glimmer-dsl-swt 4.18.6.1 up to 5.0.0.0
- Upgrade Mac package to Big Sur
- Action Menu
- Help Menu
## 1.1.0
- Upgrade to support glimmer-dsl-swt 4.17.2.0 up to 5.0.0.0
## 1.0.0
- Initial implementation
<file_sep>/README.md
# <img src="https://raw.githubusercontent.com/AndyObtiva/glimmer-cs-timer/master/images/glimmer-timer-logo.png" height=80 /> Glimmer Timer
## [<img src="https://raw.githubusercontent.com/AndyObtiva/glimmer-dsl-swt/master/images/glimmer-logo-hi-res.png" height=40 /> Glimmer Custom Shell](https://github.com/AndyObtiva/glimmer-dsl-swt/blob/master/docs/reference/GLIMMER_COMMAND.md#custom-shell-gem)
**Mac Version**

**Windows Version**
<br>
<br>
<br>

<br>
<br>
<br>
**Linux Version**
<br>
<br>
<br>

<br>
Glimmer Timer is a sample desktop GUI application built with Glimmer (JRuby Desktop Development GUI Library).
It supports a countdown timer.
[<img src="https://raw.githubusercontent.com/AndyObtiva/glimmer-cs-timer/master/images/glimmer-timer-logo.png" height=40 /> Download Mac Version](https://www.dropbox.com/s/37da66bd2qk4djf/Timer-1.2.0.dmg?dl=1)
[<img src="https://raw.githubusercontent.com/AndyObtiva/glimmer-cs-timer/master/images/glimmer-timer-logo.png" height=40 /> Download Windows Version](https://www.dropbox.com/s/f0epkjarz4l1u5a/Timer-1.1.0.msi?dl=1)
## Usage
### App Installer
Download and install the right installer for your platform:
- [Mac DMG File](https://www.dropbox.com/s/37da66bd2qk4djf/Timer-1.2.0.dmg?dl=1) (Big Sur and Older)
- [Windows MSI File](https://www.dropbox.com/s/f0epkjarz4l1u5a/Timer-1.1.0.msi?dl=1) (Windows 10)
- [Linux GEM File](https://rubygems.org/gems/glimmer-cs-timer) (All Linux Versions compatible with [SWT's use of GTK](https://www.eclipse.org/swt/faq.php))
Run by simply opening up the Timer application installed on your system.
### Command Gem
The `timer` command can be obtained from the [glimmer-cs-timer](https://rubygems.org/gems/glimmer-cs-timer) [JRuby](https://www.jruby.org/) gem and works on Mac, Windows and Linux:
- Ensure [Glimmer pre-requisites](https://github.com/AndyObtiva/glimmer-dsl-swt#pre-requisites)
- `gem install glimmer-cs-timer` (if you don't have [RVM](https://rvm.io), then prefix with `jruby -S` or run `jgem` instead)
- `timer`
## Tutorial
[How To Write a Glimmer Timer Desktop App in One Hour](https://andymaleh.blogspot.com/2020/08/writing-glimmer-timer-sample-app-in.html)
## Development
### Pre-requisites
If you would like to reuse as a library instead of a standalone application, here are the pre-requisites needed:
- [Glimmer DSL for SWT](https://github.com/AndyObtiva/glimmer-dsl-swt) application, [Glimmer](https://github.com/AndyObtiva/glimmer-dsl-swt) custom shell, or another [Glimmer](https://github.com/AndyObtiva/glimmer-dsl-swt) custom widget
- JRuby version required by [Glimmer](https://github.com/AndyObtiva/glimmer-dsl-swt)
- Java version required by [Glimmer](https://github.com/AndyObtiva/glimmer-dsl-swt)
### Setup
To setup for use in another [Glimmer](https://github.com/AndyObtiva/glimmer-dsl-swt) application, [Glimmer](https://github.com/AndyObtiva/glimmer-dsl-swt) custom shell, or another [Glimmer](https://github.com/AndyObtiva/glimmer-dsl-swt) custom widget, follow the instructions below.
#### Glimmer Application
Add the following to a Glimmer application `Gemfile`:
```ruby
gem 'glimmer-cs-timer', '1.2.0'
```
Run:
```
jruby -S bundle
```
(or just `bundle` if using RVM)
#### Glimmer Custom Shell or Glimmer Custom Widget
When reusing in a Glimmer custom shell or custom widget, you can follow the same steps for Glimmer application, and then add a require statement to your library file:
```ruby
require 'glimmer-cs-timer'
# ... more require statements follow
```
## Change Log
[CHANGELOG.md](CHANGELOG.md)
## Contributing to glimmer-cs-timer
- Check out the latest master to make sure the feature hasn't been implemented or the bug hasn't been fixed yet.
- Check out the issue tracker to make sure someone already hasn't requested it and/or contributed it.
- Fork the project.
- Start a feature/bugfix branch.
- Ensure [Glimmer pre-requisites](https://github.com/AndyObtiva/glimmer-dsl-swt#pre-requisites)
- `gem install bundler` (if you don't have [RVM](https://rvm.io), then prefix with `jruby -S` or run `jgem` instead)
- `bundle`
- Run app via `bin/timer` or `glimmer bin/glimmer-cs-timer` to ensure it works.
- Commit and push until you are happy with your contribution.
- If the changes include highly sophisticated pure model logic, then please cover it with [rspec](https://github.com/rspec/rspec) tests.
## Copyright
[MIT](https://opensource.org/licenses/MIT)
Copyright (c) 2020-2021 <NAME>.
--
[<img src="https://raw.githubusercontent.com/AndyObtiva/glimmer/master/images/glimmer-logo-hi-res.png" height=40 />](https://github.com/AndyObtiva/glimmer) Built with [Glimmer DSL for SWT](https://github.com/AndyObtiva/glimmer-dsl-swt) (JRuby Desktop Development GUI Framework)
Icon made by <a href="https://www.flaticon.com/authors/freepik" title="Freepik">Freepik</a> from <a href="https://www.flaticon.com/" title="Flaticon"> www.flaticon.com</a>
<file_sep>/lib/glimmer-cs-timer.rb
$LOAD_PATH.unshift(File.expand_path('..', __FILE__))
require 'glimmer-dsl-swt'
require 'views/glimmer/timer'
<file_sep>/bin/timer
#!/usr/bin/env ruby
require 'glimmer/launcher'
runner = File.expand_path("../glimmer-cs-timer", __FILE__)
launcher = Glimmer::Launcher.new([runner] + ARGV)
launcher.launch
| cdaadd178f18f8389e6e234b3d251e2c496684da | [
"Markdown",
"Ruby"
] | 4 | Markdown | rubycoder/glimmer-cs-timer | 6aeae4e210d01624c0c770415e7736df9c254739 | f7d30d5138f57dfbfb40c48795946042650f0b10 | |
refs/heads/master | <file_sep>require 'logger'
class NullLogger
def method_missing(*); end
end
options = {logger: false}
logger = options.fetch(:logger){Logger.new($stdout)}
unless logger
logger = NullLogger.new
end
logger
# => #<NullLogger:0x00000003b73858>
{}[:foo] || :default # => :default
{foo: nil}[:foo] || :default # => :default
{foo: false}[:foo] || :default # => :default
{}.fetch(:foo){:default} # => :default
{foo: nil}.fetch(:foo){:default} # => nil
{foo: false}.fetch(:foo){:default} # => false
<file_sep># Destructuring assignment is the process of assigning multiple variables at one time.
arr = [2, 3]
(a, b) = *arr
a # => 2
b # => 3
dep = {"hello" => ["hello.c", "foo.o", "bar.o"]}
# The first file in the dependency is the primary source file. The others are supporting libraries.
# Breaking out the parts of this dependency by assigning one piece at a time:
dep.first # => ["hello", ["hello.c", "foo.o", "bar.o"]] # Individual hash entries are represented as two element arrays.
target = dep.first.first # => "hello"
first_preq = dep.first.last.first # => "hello.c"
rest_preqs = dep.first.last[1..-1] # => ["foo.o", "bar.o"]
# A different approach:
dep = {"hello" => ["hello.c", "foo.o", "bar.o"]}
a = *dep # => [["hello", ["hello.c", "foo.o", "bar.o"]]] # We splat out the dependency into an array.
((target, (first_preq, *rest_preqs))) = *dep
target # => "hello"
first_preq # => "hello.c"
rest_preqs # => ["foo.o", "bar.o"]
<file_sep># a file called .netrc, which stores usernames and passwords for remote hosts.
machine rubytapas.com login avdi password <PASSWORD>
machine example.org login marvin password <PASSWORD>
# write our own parser for this simplified .netrc format:
open('.netrc').lines do |line|
columns = line.split
machine = columns[1]
login = columns[3]
password = columns[5]
puts "#{machine}: #{login} / #{password}"
end
# >> rubytapas.com: avdi / xyzzy
# >> example.org: marvin / plugh
# we can implicitly splat arrays into multiple variables on assignment.
open('.netrc').lines do |line|
machinekey, machine, loginkey, login, passwordkey, password = line.split
puts "#{machine}: #{login} / #{password}"
end
# >> rubytapas.com: avdi / xyzzy
# >> example.org: marvin / plugh
# But now we have a bunch of useless variables ending in -key which exist simply to be placeholders.
# We need to clarify that we don't care about any of those fields by using the variable name ignored for all of them:
open('.netrc').lines do |line|
ignored, machine, ignored, login, ignored, password = line.split
puts "#{machine}: #{login} / #{password}"
end
# As a separate method:
# If we replace our ignored variable with a variable called _, Ruby relaxes its usual rules about duplicate argument names.
def netrc_entries
open('.netrc').lines do |line|
yield(*line.split)
end
end
netrc_entries do |_, machine, _, login, _, password|
puts "#{machine}: #{login} / #{password}"
end
# >> rubytapas.com: avdi / xyzzy
# >> example.org: marvin / plugh<file_sep> text = <<END
I'm your only friend
I'm not your only friend
But I'm a little glowing friend
But really I'm not actually your friend
But I am
END
word_count = Hash.new do |hash, missing_key|
hash[missing_key] = 0
end
text.split.map(&:downcase).each do |word|
word_count[word] += 1
end
word_count
h = Hash.new { |h, k| h[k] = [] } # !> shadowing outer local variable - h
h["IPAs"] << "Victory HopDevil"
h["IPAs"] << "Weyerbacher Double Simcoe"
h["Stouts"] << "Victory Storm King"
h["Stouts"]
# => ["Victory Storm King"]
# Hash uses the same default object everywhere. It doesn't duplicate it before use.
# when we use a default block instead of a default value, the block is executed every time a default value is needed,
# thus generating a new Array object every time.
<file_sep>def greet(params)
name = user_name(params) rescue "Anonymous"
puts "Hello, #{name}"
end
#if the username is missing; we'd like to just replace it with a placeholder and keep going.
greet({})
# >> Hello, Anonymous
#if we want to examine the exception before deciding to re-raise itץ
value_or_error = {}.fetch(:some_key) rescue $! #a reference to the currently-raised exception
value_or_error # => #<KeyError: key not found: :some_key>
#rescue is an excellent way to convert exceptions into return values.
<file_sep># in the absence of differing behavior, differentiated child classes aren't needed.
# transition to using different instances to represent different units, instead of different classes.
class Quantity
attr_reader :magnitude, :unit
def initialize(magnitude, unit)
@magnitude = magnitude.to_f
@unit = unit
freeze
end
def to_s
"#{@magnitude} #{unit}"
end
def inspect
"#<#{unit}:#{@magnitude}>"
end
def +(other)
other = ensure_compatible(other)
self.class.new(@magnitude + other.to_f, unit)
end
def -(other)
other = ensure_compatible(other)
self.class.new(@magnitude - other.to_f, unit)
end
def *(other)
multiplicand = if other.is_a?(Numeric)
other
elsif other.is_a?(Quantity) && other.unit == unit
other.to_f
else
fail TypeError, "Don't know how to multiply by #{other}"
end
self.class.new(@magnitude * multiplicand, unit)
end
def <=>(other)
return nil unless other.is_a?(self.class) && other.unit == unit
magnitude <=> other.to_f
end
def Feet(value)
if value.is_a?(Quantity) && value.unit == :feet
value
else
value = Float(value)
Quantity.new(value, :feet)
end
end
def Meters(value)
if value.is_a?(Quantity) && value.unit == :meters
value
else
value = Float(value)
Quantity.new(value, :meters)
end
end
end
ConversionRatio.registry <<
ConversionRatio.new(:feet, :meters, 0.3048) <<
ConversionRatio.new(:meters, :feet, 3.28084)
# So long as some kind of object may have different sub-types, and those types affect the logic of a program,
# we need a way of representing that differentiation. The Ruby way to encode type is with a class (or module).<file_sep># It's the default return value for a Hash when the key is not found:
h = {}
h['fnord'] # => nil
def empty
# TODO
end
empty
result = if (2 + 2) == 5
"uh-oh"
end
result # => nil
# for a case statement with no matched "when" clauses and no else:
type = case :foo
when String then "string"
when Integer then "integer"
end
type # => nil
if (2 + 2) == 5
tip = "follow the white rabbit"
end
tip # => nil
# unset instance variables (f.e. typos):
@i_can_has_spelling = true
puts @i_can_haz_speling # => nil
# many ruby methods return nil to indicate failure:
[1, 2, 3].detect{|n| n == 5} # => nil
# Here's a method for fetching a password. When we call it, returns nil.
require 'yaml'
SECRETS = File.exist?('secrets.yml') && YAML.load_file('secrets.yml')
def get_password_for_user(username=ENV['USER'])
secrets = SECRETS || @secrets
entry = secrets && secrets.detect{|entry| entry['user'] == username}
entry && entry['password']
end
get_password_for_user # => nil
# And this is the fundamental problem with nil: it can mean many different things, but the meaning is always contextual.<file_sep># A doubled-up bang operator to force a value to be converted to a boolean.
code = <<EOF
bool = !!result
EOF
code.chars.chunk{|c|
case c
when /\n/ then :endline
when /[[:alpha:]]/ then :identifier
when /[\!\+\-\=\/\*]/ then :operator
when /\d/ then :number
end
}.to_a
# => [[:identifier, ["b", "o", "o", "l"]],
# [:operator, ["="]],
# [:operator, ["!", "!"]],
# [:identifier, ["r", "e", "s", "u", "l", "t"]],
# [:endline, ["\n"]]]
# A doubled-up bang shouldn't be treated as a single operator.
# Instead of "operator" we'll categorize these characters using the flag symbol :_alone.
code = <<EOF
bool = !!result
EOF
code.chars.chunk{|c|
case c
when /\n/ then :endline
when /[[:alpha:]]/ then :identifier
when /[\!\+\-\=\/\*]/ then :_alone
when /\d/ then :number
end
}.to_a
# => [[:identifier, ["b", "o", "o", "l"]],
# [:_alone, ["="]],
# [:_alone, ["!"]],
# [:_alone, ["!"]],
# [:identifier, ["r", "e", "s", "u", "l", "t"]],
# [:endline, ["\n"]]]
# Now the operator characters are chunked with the symbol :_alone.
# Receiving input chunks as an array of lines.
require 'stringio'
data = "6\r\nHello!\r\nD\r\n How are\r\n you?\r\n0\r\n"
chunked_data = StringIO.new(data)
chunks = chunked_data
.each_line("\r\n")
.chunk({chunk_num: 0, chars_left: :unknown}){
|line, state|
line.chomp!("\r\n")
if state[:chars_left] == :unknown
state[:chars_left] = Integer(line, 16)
:_separator
elsif state[:chars_left] > 0
state[:chars_left] -= line.size
state[:chunk_num]
else
state[:chunk_num] += 1
state[:chars_left] = :unknown
redo
end
}
chunks.next # => [0, ["Hello!"]]
chunks.next # => [1, [" How are", " you?"]]
<file_sep># We want to update the author with pen-name information from each of the stories.
# when we follow the author association back from one of the stories,
# we get a different object than the author object we started with—the one we are saving back to the database.
author = AM.find(1)
update_pen_names(author)
AM.store(author)
AM.find(1).pen_names
# => nil
author.object_id # => 22795980
author.stories.first.author.__get__.object_id # => 22747020
# An identity map ensures that only one object corresponds to a given record in the database at a time.
ID_MAP = {}
AM = AuthorMapper.new(ID_MAP)
SM = StoryMapper.new(ID_MAP)
#
class TeenyMapper
# ...
def initialize(id_map)
@id_map = id_map
end
def store(object)
data = object.to_h
store_row(object.class, data)
object.id = data[:id]
@id_map[[object.class, object.id]] = object #updates the identity map.
end
# ...
#fetch returns a value from the hash for the given key.
#Using an array as a Hash key shows off the fact that Ruby Hashes can accept any kind of object as a key.
def load(type, data)
@id_map.fetch([type, data[:id]]) do # we use an array of object type and ID as the key.If the identity map already contains an object matching that key, it will be returned immediately.
object = type.new #Otherwise, a new model object will be constructed, and added to the ID map before it is returned.
data.each_with_object(object) { |(key, value), o|
o[key] = value
}
@id_map[type, object.id] = object
end
end
end
author = AM.find(1)
author.object_id # => 15423800
author.stories.first.author.__get__.object_id # => 15423800
author = AM.find(1)
update_pen_names(author)
AM.store(author)
AM.find(1).pen_names
# => "<NAME>, <NAME>, <NAME>"
# we could set things up so that the identity map also functions as a cache,
# avoiding repeated database requests for objects which have already been loaded once.
before do
RubyTapas.clear_id_map
RubyTapas.base_url = Addressable::URI.parse(request.url).site.to_s
self.current_user = load_user
end<file_sep>require 'set'
a = [:first, :second, :third, :fourth]
s = Set.new(a)
# => #<Set: {:first, :second, :third, :fourth}>
s.to_a # => [:first, :second, :third, :fourth]. when we substitute a Set for our array, the implicit splatting no longer works.
x, y, z = s
x # => #<Set: {:first, :second, :third, :fourth}>
y # => nil
z # => nil
# if we rely on implicit splatting, the input must be an actual Array or something very close to one.
x, y, z = *s
x # => :first
y # => :second
z # => :third
# in the block argument list, it causes the pair to be wrapped in a second array.
def yield_pair
yield([:foo, :bar])
end
yield_pair do |*pair|
puts pair.inspect
end
# >> [[:foo, :bar]]
# If we want to work with keys and values separately, we can provide a block that takes two separate arguments.
# But if we want to work with key/value pairs as a unit, we can supply just one block argument.
<file_sep>machine rubytapas.com
login avdi
password <PASSWORD>
machine example.org
login marvin
password <PASSWORD>
# With a Struct representing a .netrc entry, we can access the username and password through method calls, or using Hash-style notation.
class Netrc
Entry = Struct.new(:login, :password) # an “entry” is a collection of unique attributes.
end
entry = Netrc::Entry.new('avdi', 'xyzzy') # implicit splatting.
entry.login # => "avdi"
entry.password # => "<PASSWORD>"
entry[:login] # => "avdi"
entry[:password] # => "<PASSWORD>"
entry[0] # => "avdi"
entry[1] # => "xyzzy"
# implicit splatting only works on arrays and “array-like” objects.
# alias entry objects' #to_a method to #to_ary. Now, Entry objects can be implicitly splatted out just like arrays.
class Netrc
Entry = Struct.new(:login, :password) do
alias_method :to_ary, :to_a
end
end
entry = Netrc::Entry.new('avdi', 'xyzzy')
login, password = entry
login # => "avdi"
password # => "<PASSWORD>"
# Now we have a class that really would make a good replacement for the old two-element-array return value.
# it adds new, meaningful accessor methods.
<file_sep>require 'open-uri'
require 'json'
def get_observation
key = ENV['WUNDERGROUND_KEY']
url = "http://api.wunderground.com/api/#{key}/conditions/q/PA/York.json"
data = open(url).read
JSON.parse(data)['current_observation']
end
def print_observation(observation)
puts "Temperature: #{observation.temp_f}F"
pressure_trend = observation.pressure_trend == "-" ? "falling" : "rising"
puts "Pressure: #{observation.pressure_mb}mb and #{pressure_trend}"
puts "Winds: #{observation.wind_string}"
end
require 'ostruct'
observation = {
'temp_f' => 49.0,
'pressure_trend' => '-',
'pressure_mb' => 981,
'wind_string' => "From the North at 3.0 MPH Gusting to 7.0 MPH"
}
os = OpenStruct.new(observation) # What we get in return is an object that has a reader method for each key in the hash.
os.temp_f # => 49.0
os.wind_string # => "From the North at 3.0 MPH Gusting to 7.0 MPH"
print_observation(get_observation)
#differences:
require 'ostruct'
s = Struct.new(:foo, :bar).new(42, 32)
os = OpenStruct.new(foo: 42, bar: 23)
s.members # => [:foo, :bar]
os.members # => nil
s.map {|value| value * 2} # => [84, 64]
os.map {|value| value * 2} # => nil
<file_sep>point = Class.new do
attr_reader :x, :y
def initialize(x, y)
@x = x
@y = y
end
end
circle = Class.new(point) do # inherit from the new class by passing the parent class to Class.new
attr_reader :radius
def initialize(x, y, radius)
super(x,y)
@radius = radius
end
end
c1 = circle.new(3,5,10)
# => #<#<Class:0x974c620>:0x974c5a8 @x=3, @y=5, @radius=10>
# when we name a class, we're simply assigning that class to a constant, just as we might assign a number or a string to a constant.
# functionally there's no difference between an all-caps constant and one that's camel-cased.
Point = Class.new do
attr_reader :x, :y
def initialize(x, y)
@x = x
@y = y
end
end
# using the class keyword is pretty much just a shorthand for creating a new class object and assigning it to a constant.
<file_sep>expr = [:*, 3, [:+, 2, 5]]
op, f1, f2 = expr
inner_op, t1, t2 = f2
op # => :*
f1 # => 3
inner_op # => :+
t1 # => 2
t2 # => 5
# By “grouping” arguments on the left side of the assignment, we can tell Ruby to assign elements of nested arrays directly to variables names.
expr = [:*, 3, [:+, 2, 5]]
op, f1, (inner_op, t1, t2) = expr
op # => :*
f1 # => 3
inner_op # => :+
t1 # => 2
t2 # => 5
# the inner addition expression has three terms. Instead of providing a variable for each term, we slurp them all up into a variable named ts.
expr = [:*, 3, [:+, 2, 5, 7]]
op, f1, (inner_op, *ts) = expr
op # => :*
f1 # => 3
inner_op # => :+
ts # => [2, 5, 7]
# splatting all of the arguments into a single array and then inspecting that array:
menu = {
'Jacked Up Turkey' => '$7.25',
'New York Mikey' => '$7.25',
'Apple Grilled Cheese' => '$5.25'
}
menu.each_with_index do |*args|
puts args.inspect
end
# >> [["Jacked Up Turkey", "$7.25"], 0]
# >> [["New York Mikey", "$7.25"], 1]
# >> [["Apple Grilled Cheese", "$5.25"], 2]
# using an intermediate variable(the pair argument):
menu = {
'Jacked Up Turkey' => '$7.25',
'New York Mikey' => '$7.25',
'Apple Grilled Cheese' => '$5.25'
}
menu.each_with_index do |pair, i|
name, price = pair
puts "#{i+1}: #{name} (#{price})"
end
# >> 1: Jacked Up Turkey ($7.25)
# >> 2: New York Mikey ($7.25)
# >> 3: Apple Grilled Cheese ($5.25
# any syntax we can use on the left side of an assignment, we can also use in an argument list.
# Instead of an intermediate pair argument, let's put a group in the block argument list:
menu = {
'Jacked Up Turkey' => '$7.25',
'New York Mikey' => '$7.25',
'Apple Grilled Cheese' => '$5.25'
}
menu.each_with_index do |(name, price), i|
puts "#{i+1}: #{name} (#{price})"
end
# >> 1: Jacked Up Turkey ($7.25)
# >> 2: New York Mikey ($7.25)
# >> 3: Apple Grilled Cheese ($5.25)
# As a result we no longer have any need of an intermediate pair variable which exists only to be broken up. <file_sep>text = <<END
Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Donec
hendrerit tempor <EMAIL> tellus. Donec pretium posuere
tellus. Proin quam nisl, tincidunt et, mattis eget, convallis nec,
purus. Cum sociis natoque penatibus et magnis dis parturient montes,
nascetur <EMAIL> ridiculus mus. Nulla posuere. Donec vitae
dolor. Nullam tristique <EMAIL> diam non turpis. Cras
placerat accumsan nulla. Nullam <EMAIL> rutrum. Nam
vestibulum accumsan nisl.
END
EMAIL_PATTERN = /\S+@\S+/i
addresses = []
while(match = EMAIL_PATTERN.match(text))
addresses << match[0]
text = match.post_match
end
addresses
# We can use the #scan method on String. This method finds every match for a given regular expression.
addresses = text.scan(EMAIL_PATTERN)
# The block will be called for each match, with the matched string as the block argument.
text.scan(EMAIL_PATTERN) do |address|
puts address
end
<file_sep>class Parent
def hello(subject="World")
puts "Hello, #{subject}"
if block_given?
yield
puts "Well, nice seeing you!"
end
end
end
class Child < Parent
def hello(subject=:default)
if subject == :default
super(&nil)
puts "How are you today?"
else
super(subject, &nil)
puts "How are you today?"
end
end
end
Child.new.hello(:default) do
puts "Hi there, Child"
end
#In order to suppress the block being passed through, we have to use the special argument &nil.
<file_sep># The string 'cherry' is being modified in-place by the #print_pie method.
def print_pie(filling)
puts filling << ' pie'
end
fruit = 'cherry'
print_pie(fruit)
fruit # => "cherry pie"
# We can clone it and pass the clone around instead.
fruit = 'cherry'
cloned_fruit = fruit.clone
print_pie(cloned_fruit)
fruit # => "cherry" # The original value remains unchanged.
# Freezing the value, getting an exception if we try to modify it:
fruit = 'cherry'
frozen_fruit = fruit.freeze
print_pie(frozen_fruit)
fruit # =>
# ~> -:2:in `print_pie': can't modify frozen String (RuntimeError)
# ~> from -:7:in `<main>'
# The simplest way to make an immutable collection is to call #freeze on an existing array:
fruits = %w(apple banana cherry damson elderberry)
fruits.freeze
fruits << 'fig' # =>
# ~> -:3:in `<main>': can't modify frozen Array (RuntimeError)
# A naturally immutable collection, using the #to_enum method to make an immutable collection:
fruits = %w(apple banana cherry damson elderberry).to_enum # enum lets us iterate over the underlying collection, but not modify it.
fruits.entries # => ["apple", "banana", "cherry", "damson", "elderberry"]
fruits << 'fig' # =>
# ~> -:3:in `<main>': undefined method `<<' for #<Enumerator> (NoMethodError)
# Making an enum from scratch:
fruits = Enumerator.new do |yielder|
yielder.yield 'apple'
yielder.yield 'banana'
yielder.yield 'cherry'
yielder.yield 'damson'
yielder.yield 'elderberry'
end
fruits.entries # => ["apple", "banana", "cherry", "damson", "elderberry"]
# Creating a new collection by changing the contents of the initial one:
fruits.class # => Enumerator
fruits.map(&:upcase) # => ["APPLE", "BANANA", "CHERRY", "DAMSON", "ELDERBERRY"] # => Array
fruits.select { |fruit| fruit.length == 6 } # => ["banana", "cherry", "damson"] # => Array
# Adding an element:
more_fruits = Enumerator.new do |yielder| # Making a modified copy of an immutable collection.
fruits.each do |fruit|
yielder.yield fruit
end
yielder.yield 'fig'
end
more_fruits.entries # => ["apple", "banana", "cherry", "damson", "elderberry"]
fruits.include?('fig') # => false
more_fruits.include?('fig') # => true
# Removing an element:
fewer_fruits = Enumerator.new do |yielder|
fruits.each do |fruit|
yielder.yield fruit unless fruit == 'cherry'
end
end
fewer_fruits.entries # => ["apple", "banana", "damson", "elderberry"]
fruits.include?('cherry') # => true
fewer_fruits.include?('cherry') # => false
# An infinite collection of even numbers:
even_numbers = Enumerator.new do |yielder|
n = 2
loop do
yielder.yield n
n += 2
end
end
even_numbers.next # => 2
even_numbers.next # => 4
even_numbers.take 10 # => [2, 4, 6, 8, 10, 12, 14, 16, 18, 20] # The enum generates more elements as they're needed.
even_or_unlucky_numbers = Enumerator.new do |yielder|
even_numbers.each do |n|
yielder.yield n
yielder.yield 13 if n == 12
end
end
even_or_unlucky_numbers.take 10 # => [2, 4, 6, 8, 10, 12, 13, 14, 16, 18]
# With cloning and freezing an Array, we can still modify the string objects it contains:
fruits = %w(apple banana cherry damson elderberry)
cloned_fruits = fruits.clone # Same with #freeze.
cloned_fruits.first.prepend 'pine'
fruits # => ["pineapple", "banana", "cherry", "damson", "elderberry"]
# An enum is regenerated every time we iterate over it:
fruits = Enumerator.new do |yielder|
yielder.yield 'apple'
yielder.yield 'banana'
yielder.yield 'cherry'
yielder.yield 'damson'
yielder.yield 'elderberry'
end
fruits.first.prepend 'pine'
fruits.entries # => ["apple", "banana", "cherry", "damson", "elderberry"]<file_sep># Numbers are immutable: they cannot be changed.
class Feet
attr_accessor :magnitude
def initialize(magnitude)
@magnitude = magnitude.to_f
end
def to_s
"#{@magnitude} feet"
end
def +(other)
Feet.new(@magnitude + other.magnitude)
end
end
class Altimeter
def initialize(value)
raise TypeError unless Feet === value
@value_in_feet = value
end
def change_by(change_amount)
raise TypeError unless Feet === change_amount
old_value = @value_in_feet
@value_in_feet.magnitude += change_amount.magnitude # the Feet class is mutable.
puts "Altitude changed from #{old_value} to #{@value_in_feet}"
end
end
require "./feet"
require "./altimeter2"
START_ALTITUDE = Feet.new(10_000) # holds a default starting altitude.
alt = Altimeter.new(START_ALTITUDE)
alt.change_by(Feet.new(500))
START_ALTITUDE # => #<Feet:0x000000017e0e90 @magnitude=10500.0>
# >> Altitude changed from 10500.0 feet to 10500.0 feet unwanted behavior, the constant's value has changed.
# Making the Feet class immutable:
class Feet
attr_reader :magnitude #
def initialize(magnitude)
@magnitude = magnitude.to_f
freeze # This tells ruby to make the object immutable.
end
def to_s
"#{@magnitude} feet"
end
def +(other)
Feet.new(@magnitude + other.magnitude)
end
def positive? # leaving a method which unintentionally updates the value in-place whenever it is called.
@magnitude = 0.0
end
end
f = Feet.new(30_000)
f.positive? # =>
# ~> -:18:in `positive?': can't modify frozen Feet (RuntimeError)
# ~> from -:23:in `<main>'
# We now have a class which ensures that we can't circumvent immutability, even accidentally.<file_sep># Returning a special string whenever the input is blank, using next:
# The argument to next becomes the return value of the current invocation of a block(instead of nil).
objects = ["house", "mouse", "", "mush", "",
"little old lady whispering 'hush'"]
result = objects.map do |o|
next "Goodnight, Moon" if o.empty? # supplying an argument to next.
"Goodnight, #{o}"
end
result.compact
# => ["Goodnight, house",
# "Goodnight, mouse",
# "Goodnight, Moon",
# "Goodnight, mush",
# "Goodnight, Moon",
# "Goodnight, little old lady whispering 'hush'"]
# A long list of filenames. We want to get all of our rules for inclusion or exclusion in one place.
# To do that, we use a series of next statements.
Dir["../**/*.mp4"].select { |f|
next false unless File.file?(f) # we skip the remaining checks using next with a false argument and it'll return false.
next false unless File.readable?(f) # skips forward if the file isn't readable.
next true if f =~ /078b-java-dregs\.mp4/ # next with a true argument skips all remaining tests and tells #select to include this entry in the results.
next false if File.size(f) == 0
next true if File.basename(f) =~ /^\d\d\d-/ # includes files matching a particular naming scheme.
}
# A method that accepts a block. It yields to the block twice, logging before and after each yield.
def yieldtwice
puts "Before first yield"
yield
puts "Before second yield"
yield
puts "After last yield"
end
yieldtwice do
puts "About to invoke next"
next # This behaves like an early return, except for a block instead of a method.
puts "Can never get here"
end
puts "After method call"
# >> Before first yield
# >> About to invoke next
# >> Before second yield
# >> About to invoke next
# >> After last yield
# >> After method call
# Using break instead of next:
def yieldtwice
puts "Before first yield"
yield
puts "Before second yield"
yield
puts "After last yield"
end
yieldtwice do
puts "About to invoke break"
break
puts "Can never get here"
end
puts "After method call"
# >> Before first yield
# >> About to invoke break
# >> After method call
# Unlike next, break doesn't just bring a yield to an early end.
# It cancels the execution of the whole method that triggered the yield.<file_sep># At this point, most of the Ruby world has embraced #raise over #fail.
# use raise when re-raising an exception.
# That way, the choice of raise instead of fail provides an extra cue to the reader that something out of the ordinary is going on.
['--require', '-r MODULE',
"Require MODULE before executing rakefile.",
lambda { |value|
begin
require value
rescue LoadError => ex
begin
rake_require value
rescue LoadError
raise ex
end
end
}
],
# In the specific case where doing an ordinary require raises a LoadError,
# this code rescues the error and then tries again to require the file using the special rake_require method.
# If that too raises a LoadError, there are no options left. And so it re-raises the exception.
# begin/raise/rescue is used for errors, and nothing else. <file_sep># This syntax doesn't always play well with larger expressions.
1..10.include?(5) # =>
# ~> -:1:in `<main>': undefined method `include?' for 10:Fixnum (NoMethodError)
# Ruby parsed the expression like this: 1..(10.include?(5)). Because of these parsing issues, we commonly see ranges included in parentheses.
# Inclusive range
(1..10).include?(10) # => true
# Exclusive range
(1...10).include?(10) # => false
# #member is the alias
(1..10).member?(10) # => true
# The threequals operator performs the same operation.
(1..10) === 5 # => true
case spaceball_one_speed
when 0...299_792_458
puts "sublight speed"
when 299_792_458
puts "light speed"
when 299_792_459...1_000_000_000
puts "ridiculous speed"
when 1_000_000_000...10_000_000_000
puts "ludicrous speed"
end
# Filtering out readings using grep.
readings = [26, 72, 9000, 8, 17, -3400]
readings.grep(0..100)
# => [26, 72, 8, 17]
# Ranges are not limited to using numbers.
("a".."z").include?("x") # => true
this_week = Time.new(2014, 7, 20)...Time.new(2014, 7, 27)
# Asking if it includes today.
this_week.include?(Time.new(2014, 7, 22))
# =>
# ~> -:2:in `each': can't iterate from Time (TypeError) # This means it has to know how to increment the start value.
# ~> from -:2:in `include?'
# ~> from -:2:in `include?'
# ~> from -:2:in `<main>'
1.succ # => 2
"a".succ # => "b"
Time.now.succ # => 2014-07-22 11:50:03 -0400 # !> Time#succ is obsolete; use time + 1
# Using #cover instead.
this_week = Time.new(2014, 7, 20)...Time.new(2014, 7, 27)
this_week.cover?(Time.new(2014, 7, 22))
# => true
# Selecting the entries for this week.
marvin_calendar = {
Time.new(2014, 7, 20) => "Mope",
Time.new(2014, 7, 22) => "Rust",
Time.new(2014, 7, 28) => "Write sad poem"
}
this_week = Time.new(2014, 7, 20)...Time.new(2014, 7, 27)
marvin_calendar.select{|date, _| this_week.cover?(date) }.values
# => ["Mope", "Rust"]
# For ranges that can be iterated, Ruby provides an #each method, along with the usual Enumerable methods.
(0..10).each do |n|
puts n
end
("a".."z").to_a
# Iterating over a range by an increment of 2, using #step (passing each (2nd) element to the block).
(0..10).step(2) do |n|
puts n
end
# >> 0
# >> 2
# >> 4
# >> 6
# >> 8
# >> 10
# Without a block, this returns an enumerator.
(0..10).step(2).to_a
# => [0, 2, 4, 6, 8, 10]
<file_sep>def hello(name)
puts "Hello, #{name}"
end
code = '
def goodbye(name)
puts "Goodbye, #{name}"
end
'
eval code
goodbye("Crow")
# >> Goodbye, Crow
# To load code more portably and robustly, we need some kind of search path of places to look for code.
# it's a global variable named $LOAD_PATH.
def try_load_file(file) # a helper method named #try_load_file.
if File.file?(file)
puts "LOAD #{file}"
eval File.read(file)
return true
else
return false
end
end
def load_lib(name) # It will take a filename as an argument.
return true if try_load_file(name) # Finds and loads the file in the current directory.
$LOAD_PATH.each do |dir| # This global variable is pre-loaded with a number of default system directories.
file = File.join(dir, name)
return if try_load_file(file)
end
fail LoadError, "Library not found: #{name}"
end
# Loading up our own library files. Expanding the path one level up from the file.
my_lib_dir = File.expand_path("..", __FILE__)
# => "/home/avdi/Dropbox/rubytapas/235-require"
# $LOAD_PATH << my_lib_dir
$LOAD_PATH.unshift(my_lib_dir) # Prepending to an array.<file_sep>class Feet; end
class Meters; end
ConversionRatio = Struct.new(:from, :to, :number) do
def self.registry
@registry ||= []
end
def self.find(from, to)
registry.detect{|ratio| ratio.from == from && ratio.to == to}
end
end
ConversionRatio.registry <<
ConversionRatio.new(Feet, Meters, 0.3048) <<
ConversionRatio.new(Meters, Feet, 3.28084)
def convert_to(target_type)
ratio = ConversionRatio.find(self.class, target_type) or # asking, not telling.
fail TypeError, "Can't convert #{self.class} to #{target_type}"
target_type.new(magnitude * ratio.number)
end
# we should tell objects what to do, rather than asking them about themselves.
class Feet; end
class Meters; end
UnitConversion = Struct.new(:from, :to) do
def self.registry
@registry ||= []
end
def self.find(from, to)
registry.detect{|ratio| ratio.from == from && ratio.to == to}
end
def call(from_value) # expected to be implemented in derived classes.
raise NotImplementedError
end
end
class RatioConversion < UnitConversion # adds a number and overrides #call.
attr_reader :number
def initialize(from, to, number)
super(from, to)
@number = number
end
def call(from_value)
from_value * number
end
end
UnitConversion.registry <<
RatioConversion.new(Feet, Meters, 0.3048) <<
RatioConversion.new(Meters, Feet, 3.28084)
def convert_to(target_type)
conversion = UnitConversion.find(self.class, target_type) or
fail TypeError, "Can't convert #{self.class} to #{target_type}"
target_type.new(conversion.call(magnitude)) # instead of reaching into the resulting object, it simply tells that object to perform its conversion.
end
# Now that we've switched from asking to telling, we easily add code to deal with temperature conversions.
require "./conversion"
class Celsius; end
class Fahrenheit; end
class BlockConversion < UnitConversion
def initialize(from, to, &block)
super(from, to)
@block = block
end
def call(from_value)
@block.call(from_value)
end
end
UnitConversion.registry << BlockConversion.new(Celsius, Fahrenheit) {
|from_value|
((from_value * 9) / 5) + 32
}
UnitConversion.find(Celsius, Fahrenheit).call(32)
# => 89
<file_sep>module LiveCell
def self.to_s() 'o' end
def self.next_generation(x, y, board)
case board.neighbors(x,y).count(LiveCell)
when 2..3 then self
else DeadCell
end
end
end
module DeadCell
def self.to_s() '.' end
def self.next_generation(x, y, board)
case board.neighbors(x,y).count(LiveCell)
when 3 then LiveCell
else self
end
end
end
[
[DeadCell, LiveCell, LiveCell, DeadCell],
# ...
]
# since this is a stateless implementation, there is no need to have individual LiveCell objects for every live cell on the board.
# And the same goes for dead cells.
<file_sep># The IO class is the basis for all input and output in Ruby.
# An IO object is effectively a wrapper around the operating systems concept of a filehandle.
# Like the File class, the Socket library subclasses from IO (such as TCPSocket or UDPSocket).
open("/dev/urandom") # => #<File:/dev/urandom>
File.superclass # => IO
require "socket"
TCPSocket.new "google.com", 80
# => #<TCPSocket:fd 7>
TCPSocket.ancestors
# => [TCPSocket, IPSocket, BasicSocket, IO, File::Constants, Enumerable, Object, JSON::Ext::Generator::GeneratorMethods::Object, Kernel, BasicObject]
# Tempfile is what is known as an IO-like object.
require "tempfile"
temp = Tempfile.new # => #<Tempfile:/tmp/20160316-13069-134v9lj>
temp.write "Hello"
temp.close
temp.open
temp.read # => "Hello"
Tempfile.ancestors
# => [Tempfile, #<Class:0x0055cfc0f224d0>, Delegator, #<Module:0x0055cfc117fe08>, BasicObject]
<file_sep># DateTime.strptime normally takes two arguments: a time string to parse, and a format specification.
# Time.strftime is the method used to convert times into strings.
require 'date'
print_format = '%-m/%-d/%Y %k:%M'
Time.new(2013, 4, 28, 9, 0).strftime(print_format)
# => "4/28/2013 9:00"
# In order to convert it to a valid strptime format to parse the same style, we only need to remove the padding modifiers from the format.
format = '%m/%d/%Y %k:%M'
DateTime.strptime('4/28/2013 9:00', format).to_time # DateTime.strptime, given no explicit time zone to parse, assumed the time was UTC.
# => 2013-04-28 05:00:00 -0400
# The sibling method called _strptime returns a hash of time components.
format = '%m/%d/%Y %k:%M'
time_parts = DateTime._strptime('4/28/2013 9:00', format)
# => {:mon=>4, :mday=>28, :year=>2013, :hour=>9, :min=>0}
# We can convert the time string to a locally-zoned Time object by breaking the string up into parts, then feeding them into Time.local:
format = '%m/%d/%Y %k:%M'
time_parts = DateTime._strptime('4/28/2013 9:00', format)
time = Time.local(
time_parts[:year],
time_parts[:mon],
time_parts[:mday],
time_parts[:hour],
time_parts[:min])
time # => 2013-04-28 09:00:00 -0400
# A gem called "chronic" figures out what time string means and gives us a Time object back:
require 'chronic'
time = Chronic.parse('4/28/2013 9:00')
time # => 2013-04-28 09:00:00 -0400<file_sep>require 'logger'
class Picard
def make_it_so(log=Logger.new($stdout))
log.info "I have instructed engineering to fix my tea kettle"
Geordi.new(log).fix_it
end
end
class Geordi
def initialize(log)
@log = log
end
def fix_it
@log.info "Reversing the flux phase capacitance!"
@log.info "Bounding a tachyon particle beam off of Data's cat!"
Barclay.new(@log).monitor_sensors
end
end
class Barclay
def initialize(log)
@log = log
end
def monitor_sensors
@log.warn "Warning, bogon levels are rising!"
end
end
Picard.new.make_it_so
# >> I, [2013-06-04T16:10:03.056063 #11351] INFO -- : I have instructed engineering to fix my tea kettle
# >> I, [2013-06-04T16:10:03.056286 #11351] INFO -- : Reversing the flux phase capacitance!
# >> I, [2013-06-04T16:10:03.056336 #11351] INFO -- : Bounding a tachyon particle beam off of Data's cat!
# >> W, [2013-06-04T16:10:03.056393 #11351] WARN -- : Warning, bogon levels are rising!
class Picard
def make_it_so(log=Logger.new($stdout))
log.info "I have instructed engineering to fix my tea kettle"
Geordi.new(log, quiet: true).fix_it
end
end
class Geordi
def initialize(log, quiet: true)
@log = log
end
# This device mimics the protocols of our usual ship's log subsystem, but simply discards its input unused.
class NullLogger
def debug(*); end
def info(*); end
def warn(*); end
def error(*); end
def fatal(*); end
end
log = NullLogger.new
puts "before"
Picard.new.make_it_so(log)
puts "after"
# >> before
# >> after
<file_sep># One way to be kind to users of your code is to include “executable documentation” of these abstract methods using stubs that raise NotImplementedError.
# LogicalCondition is an abstract class: that is, a class that must be subclassed in order to be used.
class SpaceAvailableCondition < LogicalCondition
private
def condition_holds?
!client.full?
end
end
class ItemAvailableCondition < LogicalCondition
private
def condition_holds?
!client.empty?
end
end
# NotImplementedError inherits from ScriptError, which in turn inherits directly from Exception.
begin
raise NotImplementedError
rescue
puts "Rescued error"
end
def condition_holds?
raise NotImplementedError,
"You must implement a #condition_holds? predicate that "
"tests whether the condition is currently true"
end
# Now if we try to use a LogicalCondition without subclassing and overriding #predicate_holds?, we get a helpful message telling us what we did wrong.
# users of the class can also read through the class source code and easily see the methods which are required but not provided.<file_sep># This line now does three things: it reads up to 1k of the file.
# Then it assigns the resulting string (or nil value) to the chunk variable.
book = open('../../books/confident-ruby/confident-ruby.org')
lines = 0
while chunk = book.read(1024)
lines += chunk.count("\n")
end
puts lines
# the use of the chunk variable is neatly contained within the loop it pertains to.
# If we were to ever remove the loop, we wouldn't be leaving a dangling variable behind.
# A convention that some Ruby programmers use to avoid this confusion is to always
# surround loop conditions containing inline assignment with a set of parentheses.
while(chunk = book.read(1024))
lines += chunk.count("\n")
end
# inline assignment in tests:
class SiteVisit
attr_reader :timestamp
def initialize
@timestamp = Time.now
end
end
# require 'rspec/autorun'
# describe SiteVisit do
# it 'sets timestamp to the current time' do
# expected_time = Time.new(2013, 1, 1)
# Time.stub(now: expected_time)
# visit = SiteVisit.new
# visit.timestamp.should eq(expected_time)
# end
# end
# We can save some typing in this test by inlining the assignment of the expected_time into the definition of the Time.now stub:
require 'rspec/autorun'
describe SiteVisit do
it 'sets timestamp to the current time' do
Time.stub(now: expected_time = Time.new(2013, 1, 1))
visit = SiteVisit.new
visit.timestamp.should eq(expected_time)
end
end
# for quickly naming the return value of one stubbed method, this idiom is convenient.
<file_sep>open("jabberwocky.txt").each_line.grep(/Jabberwock/) # the File#each_line method returns an enumerator over a file's lines. we pass a regex.
# => ["\"Beware the Jabberwock, my son!\n",
# "The Jabberwock, with eyes of flame,\n",
# "\"And hast thou slain the Jabberwock?\n"]
# passing a block to #grep:
open("jabberwocky.txt").each_line.grep(/Jabberwock/) do |line|
puts "MATCH: #{line}"
end
# >> MATCH: "Beware the Jabberwock, my son!
# >> MATCH: The Jabberwock, with eyes of flame,
# >> MATCH: "And hast thou slain the Jabberwock?
# we can break out early, without the expense of processing all of the elements.
open("jabberwocky.txt").each_line.grep(/Jabberwock/) do |line|
break if line =~ /slain/
puts "MATCH: #{line}"
end
# >> MATCH: "Beware the Jabberwock, my son!
# >> MATCH: The Jabberwock, with eyes of flame,
# grep can be used to match any kind of object in a collection
# it makes use of Ruby's “case-equality”, or “threequals” operator.
case object
when 23 then "the number 23" # matches an object based on value
when /foo/ then "contains foo" # a matching regex
when Float then "a floating-point number" # the class
when /2..10/ then "between 2 and 10" # a range of values
end
# comparing the argument against the collection's elements using the threequals operator:
[87, 23, 15, 74, 62, 42, 91].grep(0..50)
# => [23, 15, 42]
(0..50) === 23 # => true
(0..50) === 87 # => false
# inventing our own pattern objects for matching, since #grep implements the threequals:
# we want to find ratios based on their from and to properties.
class Feet; end
class Meters; end
ConversionRatio = Struct.new(:from, :to, :number) do
def self.registry
@registry ||= []
end
def self.find(from, to) # one way: passing a block to #detect.
registry.detect{|ratio| ratio.from == from && ratio.to == to}
end
end
ConversionRatio.registry <<
ConversionRatio.new(Feet, Meters, 0.3048) <<
ConversionRatio.new(Meters, Feet, 3.28084)
require "./ratio"
RatioPattern = Struct.new(:from, :to) do
def ===(other)
from == other.from && to == other.to
end
end
ConversionRatio.registry.grep(RatioPattern.new(Feet, Meters)) # second way: grep with an instance of this object.
# => [#<struct ConversionRatio from=Feet, to=Meters, number=0.3048>]
# third way : set up a lambda that lookds for what we want, passing it to #grep:
require "./ratio"
pattern = ->(ratio) { ratio.from == Meters && ratio.to == Feet }
ConversionRatio.registry.grep(pattern) # Ruby Proc objects alias threequals to the #call method (the pattern variable.
# => [#<struct ConversionRatio from=Meters, to=Feet, number=3.28084>]
# pattern === ConversionRatio.new(Meters, Feet, 3.28084) # => true
# with #grep we pass the pattern as an argument<file_sep># Ruby provides a shortcut for reading a file into memory and dividing it into lines all at once. It's called File.readlines.
lines = File.readlines("mail.txt", "\r\n") # Filename and an optional delimeter string that indicates linebreaks.
lines
# => ["MIME-Version: 1.0\r\n",
# "Received: by 10.112.223.164 with HTTP; Tue, 2 Sep 2014 15:17:21 -0700 (PDT)\r\n",
# "Date: Tue, 2 Sep 2014 18:17:21 -0400\r\n",
# "Delivered-To: <EMAIL>\r\n",
# "Message-ID: <<EMAIL>>\r\n",
# "Subject: TPS Reports\r\n",
# "From: <NAME> <<EMAIL>>\r\n",
# "To: <NAME> <<EMAIL>>\r\n",
# "Content-Type: text/plain; charset=UTF-8\r\n",
# "\r\n",
# "Yeah... if you could just fill out your TPS reports... that'd be great...\r\n"]
lines = File.readlines("mail.txt", "\r\n")
line = lines.shift until line == "\r\n" # removes first element of the array.
lines.join
# => "Yeah... if you could just fill out your TPS reports... that'd be great...\r\n"
# In a single line of chained message sends:
# drop_while takes a block. The block receives one line at a time, and functions as a predicate.
# The method drops items, until it finds one that fails the predicate.
# By contrast, the version using #drop_while leaves the list of lines untouched
File.readlines("mail.txt", "\r\n").drop_while{|l| l != "\r\n"}
# => ["\r\n",
# "Yeah... if you could just fill out your TPS reports... that'd be great...\r\n"]
File.readlines("mail.txt", "\r\n").drop_while{|l| l != "\r\n"}.drop(1) # #drop drops however many elements are requested.
# => ["Yeah... if you could just fill out your TPS reports... that'd be great...\r\n"]
File.readlines("mail.txt", "\r\n").drop_while{|l| l != "\r\n"}.drop(1).join
# => "Yeah... if you could just fill out your TPS reports... that'd be great...\r\n"<file_sep>class Shopper
def initialize(list)
@list = list
end
attr_accessor :list
end
shared_list = ["bread", "milk", "granola"]
stacey = Shopper.new(shared_list)
avdi = Shopper.new(shared_list)
stacey.list.concat(["swiss", "brie", "cheddar"])
stacey.list # => ["bread", "milk", "granola", "swiss", "brie", "cheddar"]
avdi.list # => ["bread", "milk", "granola", "swiss", "brie", "cheddar"]
# When we send the message #concat to the shared list, with the array of new items as an argument, the target array is updated in-place.
# We get the behavior we expect, with both shopper attributes reflecting the new contents.
# Unless you specifically want to create a new combined array rather than append to an existing one, prefer Array#concat over +=.
<file_sep>point = Struct.new(:x, :y)
point # => #<Class:0x00000004da22e0>
point.class # => Class
point.name # => nil
Point = point
Point # => Point
point # => Point
point.class # => Class
point.name # => "Point" #Ruby sets the name of the class or module to the name of the constant.
Point = Struct.new(:x, :y)
Point.new # => #<struct Point x=nil, y=nil>
Point.new(23) # => #<struct Point x=23, y=nil>
Point.new(5,7)
p = Point.new(4,5)
p[:x] # => 4
p["y"] # => 5
p[:x] = 13
p.x # => 13
<file_sep>def names
yield "Ylva"
yield "Brighid"
yield "Shifra"
yield "Yesamin"
end
# break can take an optional argument.
result = names do |name|
puts name
break name if name =~ /^S/
end
result
# Shifra
# by passing the matching name to break, we forced the names method to return that string instead of a nil.
f = open('071-break-with-value.org')
f.lines.detect do |line|
if f.lineno >= 100
break "<Not Found (Stopped at #{f.lineno}: '#{line.chomp}')>"
end
line =~ /banana/
end
# break can not only force a method to return early, it can also override the return value of the method in the process.
<file_sep># It's not a good idea to load files full of Ruby code more than once if we can avoid it.
# Where load re-evaluates a file every time it is invoked, require keeps a global list of all the files it has already processed.
# When we require some library in a Ruby program, we don't require a specific filename. We require a feature by name.
require "bigdecimal" # => true
require "bigdecimal" # => false # If it has already been loaded, require returns false.
require "bigdecimal" # If we don't require the library first, the result is false.
feature = "bigdecimal"
load_path = $LOAD_PATH.dup
if feature[0] == "."
load_path.unshift(".")
end
globs = load_path.map{|base_dir| # get a set of file glob patterns by mapping over the load path.
File.expand_path("#{feature}.*", base_dir) # .* appended, which is a file glob pattern that should match any file extension.
}
globs
files = Dir.glob(globs)
# => ["/home/avdi/.rubies/ruby-2.1.2/lib/ruby/2.1.0/x86_64-linux/bigdecimal.so"]
library = files.first
$LOADED_FEATURES
# => ["enumerator.so",
$LOADED_FEATURES.include?(library)
# => true<file_sep>objects = ["house", "mouse", "", "mush", "",
"little old lady whispering 'hush'"]
objects.each do |o|
puts "Goodnight, #{o}"
end
# >> Goodnight, house
# >> Goodnight, mouse
# >> Goodnight,
# >> Goodnight, mush
# >> Goodnight,
# >> Goodnight, little old lady whispering 'hush'
# A conditional statement around the puts:
objects.each do |o|
unless o.empty?
puts "Goodnight, #{o}"
end
end
# >> Goodnight, house
# >> Goodnight, mouse
# >> Goodnight, mush
# >> Goodnight, little old lady whispering 'hush'
# Using the next keyword to tell Ruby to skip to the next iteration:
objects.each do |o|
next if o.empty?
puts "Goodnight, #{o}"
end
# >> Goodnight, house
# >> Goodnight, mouse
# >> Goodnight, mush
# >> Goodnight, little old lady whispering 'hush'
# next skips to the next iteration, whereas break breaks completely out of the method
# that the block has been passed into (in this case: each).
# As a variable, using map and without next:
objects = ["house", "mouse", "", "mush", "",
"little old lady whispering 'hush'"]
result = objects.map do |o|
"Goodnight, #{o}"
end
# an array of strings
result
# => ["Goodnight, house",
# "Goodnight, mouse",
# "Goodnight, ",
# "Goodnight, mush",
# "Goodnight, ",
# "Goodnight, little old lady whispering 'hush'"]
# Using next:
result = objects.map do |o|
next if o.empty?
"Goodnight, #{o}"
end
result
# => ["Goodnight, house",
# "Goodnight, mouse",
# nil,
# "Goodnight, mush",
# nil,
# "Goodnight, little old lady whispering 'hush'"]
# We now know that the return value of a block invocation which is skipped by next is nil.
# Using compact to filter out all the nil entries:
result = objects.map do |o|
next if o.empty?
"Goodnight, #{o}"
end
result.compact
# => ["Goodnight, house",
# "Goodnight, mouse",
# "Goodnight, mush",
# "Goodnight, little old lady whispering 'hush'"]<file_sep># The __FILE__ variable always contains the absolute path of the file it is found in.
# The first argument to File.expand_path is the path to be expanded, and the second is the base path to start from.
__FILE__
# => /myapp/bin/hello
File.expand_path "..", __FILE__
# => /myapp/bin
File.expand_path "../..", __FILE__
# => /myapp
File.expand_path "../../cow_helpers", __FILE__
# => /myapp/cow_helpers
require File.expand_path("../../cow_helpers", __FILE__)
print CowHelpers.moo "Hello, world"<file_sep>class Point1
def self.my_new(*args, &block)
instance = allocate
instance.my_initialize(*args, &block)
instance
end
def my_initialize(x,y)
@x = x
@y = y
end
end
p = Point1.my_new(3,5)
class Point2
def self.new_cartesian(x, y)
instance = allocate
instance.initialize_cartesian(x, y)
instance
end
def self.new_polar(distance, angle)
instance = allocate
instance.initialize_cartesian(polar_to_cartesian(distance, angle))
instance
end
# ...
end
class Snowflake
class << self
private :new
end
def self.instance
@instance ||= new
end
end
Snowflake.instance # => #<Snowflake:0x00000004af4db8>
Snowflake.instance # => #<Snowflake:0x00000004af4db8>
Snowflake.new # =>
# ~> -:14:in `<main>': private method `new' called for Snowflake:Class (NoMethodError)
class RpsMove
def self.new(move)
@cache ||= {}
@cache[move] ||= super(move)
end
def initialize(move)
@move = move
end
end
RpsMove.new(:rock) # => #<RpsMove:0x00000004967428 @move=:rock>
RpsMove.new(:rock) # => #<RpsMove:0x00000004967428 @move=:rock>
<file_sep>width = 960
height = 600
recording_options = %W[-f x11grab -s #{width}x#{height} -i 0:0+800,300 -r 30]
misc_options = %W[-sameq -threads 6]
output_options = %W[screencast.mp4]
ffmpeg_flags =
recording_options +
misc_options +
output_options
system "ffmpeg", *ffmpeg_flags
<file_sep># Our deprecation warnings shouldn't be going to standard output; they should be going to the standard error stream.
def old_and_busted
$stderr.puts "#old_and_busted is deprecated. Use #new_hotness instead." # sending the puts message to the $stderr global.
puts "Hello, world"
end
def new_hotness
puts "Salutations, universe!"
end
# The warn method is like puts, only instead of defaulting to standard output, it uses $stderr.
def old_and_busted
warn "#old_and_busted is deprecated. Use #new_hotness instead."
puts "Hello, world"
end<file_sep> class PersonalAccount
def display(r)
r.name("#{first_name} #{last_name}")
r.email(email)
end
end
class CorporateAccount
def display(r)
r.name(company_name)
r.email(email)
r.tax_id(tax_id)
end
end
class TrialAccount
def display(r)
r.name("Trial Account User")
r.email(email)
end
end
# renderers
class CsvRenderer
def initialize(destination)
@csv = CSV.new(destination)
end
def method_missing(name, value=nil)
@csv << [name, value]
end
end
class HtmlAccountRenderer
def method_missing(name, value=nil)
instance_variable_set("@#{name}", value)
end
def render
<<"END"
<div class="account vcard">
<p>
Account details for:
<span class="email">#{@email}</span>
</p>
<p class="fn">#{@name}</p>
</div>
END
end
end
pa = PersonalAccount.new("Tom", "Servo", "<EMAIL>")
ca = CorporateAccount.new(
"Yoyodyne",
"<EMAIL>")
ta = TrialAccount.new("<EMAIL>")
# using the renderer
puts "Personal Account:"
renderer = HtmlAccountRenderer.new
pa.display(renderer)
puts renderer.render
puts "\nCorporate Account:"
renderer = HtmlAccountRenderer.new
ca.display(renderer)
puts renderer.render
puts "\nTrial Account:"
renderer = HtmlAccountRenderer.new
ta.display(renderer)
puts renderer.render
<file_sep>require 'delegate'
class AuditedAccount < SimpleDelegator # SimpleDelegator enables us to augment the methods we care about, and ignore all the others.
def initialize(account, audit_log)
super(account) #
@audit_log = audit_log
end
def deposit(amount)
super
@audit_log.record(number, :deposit, amount, balance_cents)
end
def withdraw(amount)
super
@audit_log.record(number, :withdraw, amount, balance_cents)
end
end
# Thnaks to the delegate library, if we compare the raw account and the decorated account, the result is true.
# This helps ensure that delegator objects are usable as drop-in replacements for the raw object in as many contexts as possible.
require "./bank_account"
require "./audit_log"
require "./audited_account"
account = BankAccount.new(123456)
log = AuditLog.new
aa = AuditedAccount.new(account, log)
account.number # => 123456
aa.number # => 123456
aa == account # => true
# Defining a variant of AuditedAccount using DelegateClass(invoking a global method):
require "./bank_account"
require "delegate"
DelegateClass(BankAccount) # Providing the name of the class we intend to decorate(generates a new base class at runtime).
# => #<Class:0x007f9daf5f9c50>
require "delegate"
require "./bank_account"
class AuditedAccount2 < DelegateClass(BankAccount) # Instances of this class will respond to the number attribute(because it generates proxy methods ahead of time).
def initialize(account, audit_log)
super(account)
@audit_log = audit_log
end
def deposit(amount)
super
@audit_log.record(number, :deposit, amount, balance_cents)
end
def withdraw(amount)
super
@audit_log.record(number, :withdraw, amount, balance_cents)
end
end
require "./bank_account"
require "./audit_log"
require "./audited_account"
require "./audited_account2"
account = BankAccount.new(123456)
log = AuditLog.new
aa1 = AuditedAccount.new(account, log)
aa2 = AuditedAccount2.new(account, log)
aa1.number # => 123456
aa2.number # => 123456
AuditedAccount.instance_methods.include?(:number)
# => false
AuditedAccount2.instance_methods.include?(:number)
# => true
require "./bank_account.rb"
class InterestAccount < BankAccount
def interest_rate
1.3
end
end
require "./interest_account"
require "./audit_log"
require "./audited_account2"
account = InterestAccount.new(123456)
aa = AuditedAccount2.new(account, AuditLog.new) # DelegateClass-generated classes just fall back to dynamic method discovery when forwarding to a method they don't know about.
account.interest_rate # => 1.3
aa.interest_rate # => 1.3
# If we know exactly the class we plan on wrapping, we might as well use DelegateClass (better performance and introspection).
# To minimize dependencies or to decorate a range of similar classes, SimpleDelegator is an easy choice.<file_sep>a1 = [:first, :second, :third, :fourth]
a2 = [:before, a1, :after]
a2.flatten
# => [:before, :first, :second, :third, :fourth, :after]
a2 = [:before, *a1, :after]
# => [:before, :first, :second, :third, :fourth, :after]
# We prefix a1 with an asterisk, telling Ruby to “splat it out” into its component elements rather than inserting the array object.
a1 = [:first, :second, :third, :fourth]
x, y, z = *a1
x # => :first
y # => :second
z # => :third
a1 = [:first, :second, :third, :fourth]
*x, y, z = *a1
x # => [:first, :second]
y # => :third
z # => :fourth
a1 = [:first, :second, :third, :fourth]
first, *rest = *a1
first # => :first
rest # => [:second, :third, :fourth]
# with methods:
def sum3(x, y, z)
x + y + z
end
triangle = [90, 30, 60]
sum3(*triangle) # => 180
def random_draw(num_times, num_draws)
num_times.times do
draws = num_draws.times.map { rand(10) }
yield(*draws)
end
end
random_draw(5, 3) do |first, second, third| # five times, 3 numbers.
puts "#{first} #{second} #{third}"
end
# >> 9 5 3
# >> 3 8 1
# >> 4 7 6
# >> 2 1 3
# >> 8 3 3
# By applying a splat to the last parameter, we force it to “soak up” all remaining arguments (if any) into a new array.
def random_draw(num_times, num_draws)
num_times.times do
draws = num_draws.times.map { rand(10) }
yield(*draws)
end
end
random_draw(5, 3) do |first, *rest|
puts "#{first}; #{rest.inspect}"
end
# >> 7; [7, 0]
# >> 6; [6, 9]
# >> 6; [6, 2]
# >> 2; [2, 3]
# >> 3; [2, 2]
<file_sep>
puts "__FILE__: #{__FILE__}"
puts "expand_path: #{File.expand_path("..", __FILE__)}"
puts "__dir__: #{__dir__}"
# Moving the file to a subdirectory and then running it:
$ mkdir foo
$ mv whereami.rb foo
$ ruby foo/whereami.rb
__FILE__: foo/whereami.rb
expand_path: /home/avdi/Dropbox/rubytapas/245-current-dir/foo
__dir__: /home/avdi/Dropbox/rubytapas/245-current-dir/foo
# Evaluating from inside another Ruby program:
# The expand_path version winds up showing the current working directory, rather than the location of the source file.
# __dir__ has a nil value when Ruby doesn't know what file the current code is from.
eval File.read("foo/whereami.rb")
# >> __FILE__: (eval)
# >> expand_path: /home/avdi/Dropbox/rubytapas/245-current-dir
# >> __dir__:
# __dir__ is more honest, while the expand_path version is misleading.<file_sep>@names = %w[<NAME>]
def names
yield @names.shift # shift returns the first element of self and removes it.
yield @names.shift
yield @names.shift
yield @names.shift
end
enum = to_enum(:names)
@names = %w[<NAME>]
enum.rewind
enum.next
# we can drive the method's execution forward as needed by calling #next on the Enumerator.
enum.with_index do |name, index|
puts "#{index}: #{name}"
end
# An Enumerator takes any method that yields values to a block, and turns it into a lazy, iterable object
# which supports all the convenient methods of Enumerable.
<file_sep># This gives us a Proc object, which we can then assign to variables and pass around.
# When we want the code to execute, we send it the #call method.
greeter = Proc.new do |name|
puts "Hello, #{name}"
end
greeter # => #<Proc:0x0000000410cd00@-:1>
greeter.call("Ylva")
# >> Hello, Ylva
greeter["Kashti"]
greeter.("Josh")
# the lambda version adds the word “lambda” when inspected. They both execute when called.
# One difference is that the lambda version is pickier about arguments.
# The basic Proc just throws away extra arguments, whereas the lambda version reports an error.
greeter_p = proc do |name|
puts "Hello, #{name}"
end
greeter_l = lambda do |name|
puts "Hello, #{name}"
end
greeter_p # => #<Proc:0x0000000338efe8@-:1>
greeter_l # => #<Proc:0x0000000338efc0@-:4 (lambda)>
greeter_p.call("Lily")
greeter_l.call("Stacey")
# There is also an extra short form for writing lambdas, which is sometimes referred to as “stabby lambda” syntax.
# method-style parentheses, instead of vertical pipes.
greeter_l = ->(name) {
puts "Hello, #{name}"
}
greeter_l # => #<Proc:0x000000031fba50@-:1 (lambda)>
#Every Ruby method can implicitly receive a proc argument, which is called using the yield keyword.
def each_child
yield "Lily"
yield "Josh"
yield "Kashti"
yield "Ebba"
yield "Ylva"
end
each_child do |name|
puts "Hello, #{name}!"
end
# >> Hello, Lily!
# >> Hello, Josh!
# >> Hello, Kashti!
# >> Hello, Ebba!
# >> Hello, Ylva!
<file_sep># We use the Hash#fetch method to get the value or provide a default if it is not present.
def order_burger(customer_name, options={})
has_cheese = options.fetch(:cheese) # This line will raise a KeyError if no :cheese option is specified.
toppings = []
toppings << :onions if options.fetch(:onions) { true }
toppings << :bacon if options.fetch(:bacon) { false }
patty_type = options.fetch(:patty) { :beef }
print "That's one #{patty_type} #{has_cheese && "cheese"}burger"
print " with #{toppings.join(', ')}"
print " for #{customer_name}.\n"
end
order_burger("Grimm", cheese: true, bacon: true, onions: false)
# >> That's one beef cheeseburger with bacon for Grimm.
# Ruby lets us omit the braces around a Hash argument if it's the last argument passed to a method. keyword-style arguments.
def order_burger(customer_name, options={})
patty_type = options.delete(:patty) { :beef } # checks that only known options are specified.
has_cheese = options.delete(:cheese)
toppings = []
toppings << :onions if options.delete(:onions) { true }
toppings << :bacon if options.delete(:bacon) { false }
if options.any? # checks if there are any options left in the hash.
raise ArgumentError, "Unrecognized option(s): #{options}"
end
print "That's one #{patty_type} #{has_cheese && "cheese"}burger"
print " with #{toppings.join(', ')}"
print " for #{customer_name}.\n"
end
order_burger("Grimm", cheese: true, bacon: true, onion: false)
# ~> -:8:in `order_burger': Unrecognized option(s): {:onion=>false} (ArgumentError)
# the cheese keyword is a required option, so we don't supply a default for it.
def order_burger(customer_name, patty: :beef, cheese:, onions: true, bacon: false)
toppings = []
toppings << :onions if onions
toppings << :bacon if bacon
print "That's one #{patty} #{cheese ? "cheese" : ""}burger"
print " with #{toppings.join(', ')}"
print " for #{customer_name}.\n"
end
# With just a “cheese” option, it supplies the defaults for patty type and toppings.
order_burger("Grimm", cheese: true)
# >> That's one beef cheeseburger with onions for Grimm.<file_sep># exceptions for flow control? That's where code raises an exception, not to signal an error,
# but in order to make an early escape from a deeply nested chain of method calls.
class ExcerptParser < Nokogiri::XML::SAX::Document
class DoneException < StandardError; end
# ...
def self.get_excerpt(html, length, options)
me = self.new(length,options)
parser = Nokogiri::HTML::SAX::Parser.new(me)
begin
parser.parse(html) unless html.nil?
rescue DoneException #
# we are done
end
me.excerpt
end
# ...
def characters(string, truncate = true, count_it = true, encode = true)
return if @in_quote
encode = encode ? lambda{|s| ERB::Util.html_escape(s)} : lambda {|s| s}
if count_it && @current_length + string.length > @length
length = [0, @length - @current_length - 1].max
@excerpt << encode.call(string[0..length]) if truncate
@excerpt << "…"
@excerpt << "</a>" if @in_a
raise DoneException.new
end
@excerpt << encode.call(string)
@current_length += string.length if count_it
end
end
# in order to get a page excerpt, the ExcerptParser only needs to collect text from the HTML page until it reaches the desired excerpt length.
# this class needs to be able to “break out” of the parser and signal the code that started the parse that it has all it needs (by raising an exception).
class ExcerptParser < Nokogiri::XML::SAX::Document
# ...
def self.get_excerpt(html, length, options)
me = self.new(length,options)
parser = Nokogiri::HTML::SAX::Parser.new(me)
catch(:done) do #If at any point code executed within this block “throws” the symbol :done, catch will do exactly what it sounds like – catch the symbol.
parser.parse(html) unless html.nil? #Execution will then proceed onward from the end of the catch block.
end
me.excerpt
end
# ...
def characters(string, truncate = true, count_it = true, encode = true)
return if @in_quote
encode = encode ? lambda{|s| ERB::Util.html_escape(s)} : lambda {|s| s}
if count_it && @current_length + string.length > @length
length = [0, @length - @current_length - 1].max
@excerpt << encode.call(string[0..length]) if truncate
@excerpt << "…"
@excerpt << "</a>" if @in_a
throw :done #We change the raise to a throw. When the ExcerptParser finds it has enough text for an excerpt, it “throws” the :done symbol.
end
@excerpt << encode.call(string)
@current_length += string.length if count_it
end
end
# the code no longer suggests an error condition where there actually is none.
# By contrast, with a begin/rescue/end block we have to skip down to the end of code in question to see what errors might occur.
# This is fine for errors, but less fine for perfectly normal early returns.<file_sep>require 'pp'
[0,1,2,3,4,5,6,7,8,9].each_slice(2) do |slice|
pp slice
end
# >> [0, 1]
# >> [2, 3]
# >> [4, 5]
# >> [6, 7]
# >> [8, 9]
# But if we call it without a block, it returns an Enumerator.
# chaining enumerable operations:
require 'pp'
sums = [0,1,2,3,4,5,6,7,8,9].each_slice(2).map do |slice|
slice.reduce(:+)
end
sums # => [1, 5, 9, 13, 17]
# how to duplicate this behavior in our own methods:
def names
return to_enum(:names) unless block_given? # return to_enum(__callee__) to ensure that if we ever change the name of the method the call to #to_enum will continue to work.
yield "Ylva"
yield "Brighid"
yield "Shifra"
yield "Yesamin"
end
names # => #<Enumerator: main:names>
names.to_a # => ["Ylva", "Brighid", "Shifra", "Yesamin"]
<file_sep>headers = <<END
Accept: */*
Set-Cookie: foo=42
Set-Cookie: bar=23
END
def parse_headers(headers)
headers.lines.reduce({}) do |result, line|
name, value = line.split(":")
result.merge(name.strip => value.strip)
end
end
p parse_headers(headers)
# A better implementation might collect value of repeated headers into an array.
# We can supply an optional block to the #merge method. When there's a key collision, this block will be used to determine how to resolve it.
def parse_headers(headers)
headers.lines.reduce({}) do |result, line|
name, value = line.split(":")
result.merge(name.strip => value.strip) {
|key, left, right| #
Array(left) + Array(right)
}
end
end
p parse_headers(headers)
accounting = {
"burger" => 3,
"cheesesteak" => 1,
"veggie wrap" => 2
}
engineering = {
"burger" => 2,
"gyro" => 3
}
marketing = {
"burger" => 1,
"veggie wrap" => 2,
"gyro" => 1
}
order = [accounting, engineering, marketing].reduce({}) {
|result, dept|
result.merge(dept) {
|key, left, right|
left + right
}
}
order
# => {"burger"=>6, "cheesesteak"=>1, "veggie wrap"=>4, "gyro"=>4}
#In the block we just ignore the right-hand value entirely(trance), and always use the left-hand one(drum&bass).
options = { genre: "drum&bass" }
full_options = options.merge(genre: "trance", bpm: 140){|_, left, _| left}
full_options
# => {:genre=>"drum&bass", :bpm=>140}
# by passing a block to #merge, we can easily achieve any Hash-merging strategy we can think up.<file_sep>def names
yield "Ylva"
yield "Brighid"
yield "Shifra"
yield "Yesamin"
ensure
puts "Grimm"
end
names do |name|
puts name
break if name =~ /^S/
end
# Ylva
# Brighid
# Shifra
# Grimm
# break is not just for loops and iteration. It can force an early return from any method that yields.
# While break can end a method's execution early, it still respects ensure blocks.
| fa44f4dce73386a00b6e9b872650182f478871a8 | [
"Ruby"
] | 51 | Ruby | m-gb/rubytapas | 519860d7696eedea4a4a7524ae147198f666d3a0 | 2e89742a7527144b3c6f258e4725ce756b0bcc43 | |
refs/heads/master | <repo_name>HelloNYC2016/Computer-Vision<file_sep>/Project 1/ImageProcess/src/Demo.java
import java.awt.*;
import java.awt.image.*;
import java.io.*;
import java.awt.event.*;
import javax.swing.*;
import javax.imageio.*;
import javax.swing.event.*;
public class Demo extends JApplet {
Image edgeDetectedImage, lineDetectedImage, parallelogramDetectedImage;
MediaTracker tracker = null;
PixelGrabber grabber = null;
int width = 0, height = 0;
String fileNames[] = { "TestImage1c.jpg", "TestImage2c.jpg", "TestImage3.jpg"};
// slider constraints
static final int TH_MIN = 0;
static final int TH_MAX = 255;
static final int TH_INIT = 40;
int threshold = TH_INIT;
int minimumLengthOfLines = 100;
boolean thresholdActive = false;
boolean isOverlaid = false;
int imageNumber = 0;
public int orig[] = null;
Image image[] = new Image[fileNames.length];
Image displayImage[] = new Image[fileNames.length];
JPanel controlPanel, imagePanel;
JLabel origLabel, edgeDetectedLabel, lineDetectedLabel, parallelogramDetectedLabel, comboLabel,
minimumLengthOfLinesLabel;
JSlider thresholdSlider, lengthSlider;
JButton thresholding;
JComboBox imSel;
static Sobel edgedetector;
static LineHough linedetector;
static FindParallelogram parallelogramDetector;
// Applet init function
public void init() {
tracker = new MediaTracker(this);
for (int i = 0; i < fileNames.length; i++) {
image[i] = getImage(this.getCodeBase(), fileNames[i]);
tracker.addImage(image[i], i);
}
try {
tracker.waitForAll();
} catch (InterruptedException e) {
System.out.println("error: " + e);
}
Container cont = getContentPane();
cont.removeAll();
cont.setLayout(new BorderLayout());
controlPanel = new JPanel();
controlPanel.setLayout(new GridLayout(2, 4, 15, 0));
imagePanel = new JPanel();
// row 1, col 1
comboLabel = new JLabel("SELECT AN IMAGE");
comboLabel.setHorizontalAlignment(JLabel.CENTER);
controlPanel.add(comboLabel);
// row 1, col 2
thresholding = new JButton("Thresholding Off");
thresholding.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if (thresholdActive == true) {
thresholdActive = false;
thresholding.setText("Thresholding Off");
thresholdSlider.setEnabled(false);
} else {
thresholdActive = true;
thresholding.setText("Threshold Value = " + threshold);
thresholdSlider.setEnabled(true);
}
processImage();
}
});
controlPanel.add(thresholding);
// row 1, col 3
minimumLengthOfLinesLabel = new JLabel("Minimum Length of Line detected = " + minimumLengthOfLines);
minimumLengthOfLinesLabel.setHorizontalAlignment(JLabel.CENTER);
controlPanel.add(minimumLengthOfLinesLabel);
// row 2, col 1
imSel = new JComboBox(fileNames);
imageNumber = imSel.getSelectedIndex();
imSel.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
imageNumber = imSel.getSelectedIndex();
// change size
width = image[imageNumber].getWidth(null);
height = image[imageNumber].getHeight(null);
origLabel.setIcon(
new ImageIcon(image[imageNumber].getScaledInstance(width / 3, -1, Image.SCALE_SMOOTH)));
processImage();
}
});
controlPanel.add(imSel);
// row 2, col 2
thresholdSlider = new JSlider(JSlider.HORIZONTAL, TH_MIN, TH_MAX, TH_INIT);
thresholdSlider.addChangeListener(new ChangeListener() {
@Override
public void stateChanged(ChangeEvent e) {
// TODO Auto-generated method stub
JSlider source = (JSlider) e.getSource();
if (!source.getValueIsAdjusting()) {
threshold = source.getValue();
thresholding.setText("Threshold Value = " + threshold);
processImage();
}
}
});
thresholdSlider.setMajorTickSpacing(40);
thresholdSlider.setMinorTickSpacing(10);
thresholdSlider.setPaintTicks(true);
thresholdSlider.setPaintLabels(true);
controlPanel.add(thresholdSlider);
// row 2, col 3
lengthSlider = new JSlider(JSlider.HORIZONTAL, 0, 255, minimumLengthOfLines);
lengthSlider.addChangeListener(new ChangeListener() {
@Override
public void stateChanged(ChangeEvent e) {
// TODO Auto-generated method stub
JSlider source = (JSlider) e.getSource();
if (!source.getValueIsAdjusting()) {
minimumLengthOfLines = source.getValue();
minimumLengthOfLinesLabel.setText("Minimum Length of Line detected = " + minimumLengthOfLines);
processImage();
}
}
});
lengthSlider.setMajorTickSpacing(40);
lengthSlider.setMinorTickSpacing(10);
lengthSlider.setPaintTicks(true);
lengthSlider.setPaintLabels(true);
controlPanel.add(lengthSlider);
// default size
width = image[imageNumber].getWidth(null);
height = image[imageNumber].getHeight(null);
origLabel = new JLabel("Original Image",
new ImageIcon(image[imageNumber].getScaledInstance(width / 3, -1, Image.SCALE_SMOOTH)), JLabel.CENTER);
origLabel.setVerticalTextPosition(JLabel.BOTTOM);
origLabel.setHorizontalTextPosition(JLabel.CENTER);
origLabel.setForeground(Color.blue);
imagePanel.add(origLabel);
edgeDetectedLabel = new JLabel("Edge Detected",
new ImageIcon(image[imageNumber].getScaledInstance(width / 3, -1, Image.SCALE_SMOOTH)), JLabel.CENTER);
edgeDetectedLabel.setVerticalTextPosition(JLabel.BOTTOM);
edgeDetectedLabel.setHorizontalTextPosition(JLabel.CENTER);
edgeDetectedLabel.setForeground(Color.blue);
imagePanel.add(edgeDetectedLabel);
lineDetectedLabel = new JLabel("Hough Line Image",
new ImageIcon(image[imageNumber].getScaledInstance(width / 3, -1, Image.SCALE_SMOOTH)), JLabel.CENTER);
lineDetectedLabel.setVerticalTextPosition(JLabel.BOTTOM);
lineDetectedLabel.setHorizontalTextPosition(JLabel.CENTER);
lineDetectedLabel.setForeground(Color.blue);
imagePanel.add(lineDetectedLabel);
cont.add(controlPanel, BorderLayout.NORTH);
cont.add(imagePanel, BorderLayout.CENTER);
processImage();
}
public int[] threshold(int[] original, int value) {
for (int x = 0; x < original.length; x++) {
if ((original[x] & 0xff) >= value)
// foreground is set to black
original[x] = 0xff000000;
else
// background is set to white
original[x] = 0xffffffff;
}
return original;
}
private int[] overlayImage(int[] input) {
int[] myImage = new int[width * height];
PixelGrabber grabber = new PixelGrabber(image[imageNumber], 0, 0, width, height, myImage, 0, width);
try {
grabber.grabPixels();
} catch (InterruptedException e2) {
System.out.println("error: " + e2);
}
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
if ((input[y * width + x] & 0xff) > 0)
myImage[y * width + x] = 0xffff0000;
}
}
return myImage;
}
private void processImage() {
orig = new int[width * height];
PixelGrabber grabber = new PixelGrabber(image[imageNumber], 0, 0, width, height, orig, 0, width);
try {
grabber.grabPixels();
} catch (InterruptedException e2) {
System.out.println("error: " + e2);
}
thresholdSlider.setEnabled(false);
thresholding.setEnabled(false);
imSel.setEnabled(false);
edgeDetectedLabel.setEnabled(false);
lengthSlider.setEnabled(false);
edgedetector = new Sobel();
linedetector = new LineHough();
new Thread() {
public void run() {
// detect edge
edgedetector.init(orig, width, height);
orig = edgedetector.process();
if (thresholdActive == true)
orig = threshold(orig, threshold);
edgeDetectedImage = createImage(new MemoryImageSource(width, height, orig, 0, width));
// detect line
if (thresholdActive == true) {
linedetector.init(orig, width, height);
linedetector.setLength(minimumLengthOfLines);
orig = linedetector.process();
lineDetectedImage = createImage(new MemoryImageSource(width, height, overlayImage(orig), 0, width));
} else {
lineDetectedImage = createImage(new MemoryImageSource(width, height, orig, 0, width));
}
SwingUtilities.invokeLater(new Runnable() {
public void run() {
edgeDetectedLabel.setIcon(
new ImageIcon(edgeDetectedImage.getScaledInstance(width / 3, -1, Image.SCALE_SMOOTH)));
lineDetectedLabel.setIcon(
new ImageIcon(lineDetectedImage.getScaledInstance(width / 3, -1, Image.SCALE_SMOOTH)));
if (thresholdActive == true) {
thresholdSlider.setEnabled(true);
}
thresholding.setEnabled(true);
imSel.setEnabled(true);
edgeDetectedLabel.setEnabled(true);
lengthSlider.setEnabled(true);
}
});
// write image to file
edgeDetectedLabel.addMouseListener(new MouseAdapter() {
public void mouseClicked(MouseEvent event) {
try {
// Create a buffered image with transparency
BufferedImage bimage = new BufferedImage(edgeDetectedImage.getWidth(null),
edgeDetectedImage.getHeight(null), BufferedImage.TYPE_BYTE_GRAY);
// Draw the image on to the buffered image
Graphics2D bGr = bimage.createGraphics();
bGr.drawImage(edgeDetectedImage, 0, 0, null);
bGr.dispose();
String filename = fileNames[imSel.getSelectedIndex()];
filename = filename.substring(0, filename.lastIndexOf('.'));
String path;
if (thresholdActive == true) {
path = String.format("/Users/shingshing/desktop/result/%s_T=%d.jpg", filename,
threshold);
} else {
path = String.format("/Users/shingshing/desktop/result/%s_edge_detected.jpg", filename);
}
ImageIO.write(bimage, "jpg", new File(path));
} catch (IOException e) {
System.out.println(e);
}
}
});
}
}.start();
}
}<file_sep>/Project 1/ImageProcess/src/FindParallelogram.java
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import data.Line;
import data.Parallelogram;
import data.Point;
public class FindParallelogram {
int width;
int height;
List<Line> lines;
List<Parallelogram> parallelograms;
Map<Point, Integer> points;
public FindParallelogram(int width, int height, List<Line> lines) {
super();
this.width = width;
this.height = height;
this.lines = lines;
}
public void findParallelogram() {
//HashMap is used to reduce duplicate find
points = new HashMap<>();
List<Parallelogram> parallelograms = new ArrayList<>();
//For each line we find with Hough Transform
for (int i = 0; i < lines.size(); i++) {
Line line = lines.get(i);
List<Line> parallels = findParallel(line);
List<Line> crossLines = findCrossLine(line);
for (Line parallel : parallels) {
for (Line crossLine : crossLines) {
Point p1 = findIntersection(crossLine, line);
Point p2 = findIntersection(crossLine, parallel);
//The intersection has to be valid and not already been added to the map
if (isPointValid(p1) && isPointValid(p2) && !points.containsKey(p1) && !points.containsKey(p2)) {
points.put(p1, 1);
points.put(p2, 1);
List<Line> parallelsOfCrossLine = findParallel(crossLine);
for (Line parallelOfCrossLine : parallelsOfCrossLine) {
Point p3 = findIntersection(parallelOfCrossLine, line);
Point p4 = findIntersection(parallelOfCrossLine, parallel);
if (isPointValid(p3) && isPointValid(p4) && !points.containsKey(p3) && !points.containsKey(p4)) {
parallelograms.add(new Parallelogram(p1, p2, p3, p4));
points.put(p3, 1);
points.put(p4, 1);
}
}
}
}
}
}
for (Parallelogram parallelogram : parallelograms) {
System.out.println(parallelogram.toString());
}
}
private Point findIntersection(Line line1, Line line2) {
int angle = Math.abs(line1.angle - line2.angle);
//We don't find intersections for two lines that are almost parallel
if (angle > 10 && angle < 350) {
int r1 = line1.r, r2 = line2.r;
int a1 = line1.angle, a2 = line2.angle;
int x = (int) ((r2*Math.sin(((a1)*Math.PI)/180) - r1*Math.sin(((a2)*Math.PI)/180))
/(Math.cos(((a2)*Math.PI)/180) * Math.sin(((a1)*Math.PI)/180) - Math.cos(((a1)*Math.PI)/180)*Math.sin(((a2)*Math.PI)/180)));
int y = (int) ((r2*Math.cos(((a1)*Math.PI)/180) - r1*Math.cos(((a2)*Math.PI)/180))
/(Math.cos(((a1)*Math.PI)/180) * Math.sin(((a2)*Math.PI)/180) - Math.cos(((a2)*Math.PI)/180)*Math.sin(((a1)*Math.PI)/180)));
Point point = new Point(x, y);
return point;
} else {
return new Point(-1, -1);
}
}
private boolean isPointValid(Point point) {
//intersection must me in the image
if (point.x < width && point.x >= 0 && point.y >= 0 && point.y < height) return true;
else return false;
}
//If two lines almost coincide, they are not regarded as parallel here
//There should be some distance between two parallel lines, the threshold here is 50
private List<Line> findParallel(Line line) {
List<Line> parallels = new ArrayList<>();
for (int i = 0; i < lines.size(); i++) {
int diff = Math.abs(line.angle - lines.get(i).angle);
if (((diff >= 0 && diff <= 4) || (diff <= 182 && diff >= 178))
&& Math.abs(line.r - lines.get(i).r) > 50)
parallels.add(lines.get(i));
}
return parallels;
}
private List<Line> findCrossLine(Line line) {
List<Line> crossLines = new ArrayList<>();
for (int i = 0; i < lines.size(); i++) {
Point intersection = findIntersection(line, lines.get(i));
if (isPointValid(intersection)) {
crossLines.add(lines.get(i));
}
}
return crossLines;
}
}
<file_sep>/README.md
# Computer-Vision
Project 1: Parallelograms Detection
Project 2: Face Recognition
<file_sep>/Project 1/ImageProcess/src/LineHough.java
import java.lang.Math;
import java.util.ArrayList;
import java.util.List;
import data.Line;
public class LineHough {
int[] input;
int[] output;
int width;
int height;
int[] acc;
int minimumLengthOfLine;
List<Line> results;
FindParallelogram parallelogramDetector;
public void init(int[] inputIn, int widthIn, int heightIn) {
width = widthIn;
height = heightIn;
input = new int[width * height];
output = new int[width * height];
input = inputIn;
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
output[y * width + x] = 0xff000000;
}
}
}
public void setLength(int len) {
minimumLengthOfLine = len;
}
// hough transform for lines (polar), returns the accumulator array
public int[] process() {
// for polar we need accumulator of 360 degress * the longest length in
// the image
int rmax = (int) Math.sqrt(width * width + height * height);
acc = new int[rmax * 360];
int r;
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
// foreground 0xff000000
if ((input[y * width + x] & 0xff) == 0) {
for (int angle = 0; angle < 360; angle++) {
r = (int) (x * Math.cos(((angle) * Math.PI) / 180) + y * Math.sin(((angle) * Math.PI) / 180));
if ((r > 0) && (r <= rmax))
acc[r * 360 + angle] = acc[r * 360 + angle] + 1;
}
}
}
}
// now normalise to 255 and put in format for a pixel array
int max = 0;
// Find max acc value
for (r = 0; r < rmax; r++) {
for (int angle = 0; angle < 360; angle++) {
if (acc[r * 360 + angle] > max) {
//System.out.println("r =" + r + ", theta = " + angle * 3 + 1);
max = acc[r * 360 + angle];
}
}
}
// System.out.println("Max :" + max);
// Normalise all the values
int value;
for (r = 0; r < rmax; r++) {
for (int angle = 0; angle < 360; angle++) {
value = (int) (((double) acc[r * 360 + angle] / (double) max) * 255.0);
acc[r * 360 + angle] = 0xff000000 | (value << 16 | value << 8 | value);
}
}
threshold();
//find parallelograms
parallelogramDetector = new FindParallelogram(width, height, new ArrayList<Line>(results));
parallelogramDetector.findParallelogram();
return output;
}
private void threshold() {
// for polar we need accumulator of 360degress * the longest length in
// the image
int rmax = (int) Math.sqrt(width * width + height * height);
results = new ArrayList<>();
for (int r = 0; r < rmax; r++) {
for (int angle = 0; angle < 360; angle++) {
int value = (acc[r * 360 + angle] & 0xff);
// if its higher than the threshold add it
if (value >= minimumLengthOfLine) {
Line line = new Line(value, r, angle);
results.add(line);
}
}
}
for (Line line : results) {
drawPolarLine(line.value, line.r, line.angle);
}
}
// draw a line given polar coordinates (and an input image to allow drawing
// more than one line)
private void drawPolarLine(int value, int r, int angle) {
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
int temp = (int) (x * Math.cos(((angle) * Math.PI) / 180) + y * Math.sin(((angle) * Math.PI) / 180));
if ((temp - r) == 0)
output[y * width + x] = 0xff000000 | (value << 16 | value << 8 | value);
}
}
}
} | 13252cd7b060a192d7230ce6a8bd3577cd320ec7 | [
"Markdown",
"Java"
] | 4 | Java | HelloNYC2016/Computer-Vision | c5a656c54a46f841476149c4e35249dc7aaf2ad5 | dfdf0d040795e56ec5b5f62135bd91df1154928a | |
refs/heads/master | <repo_name>Dia-Bhavsar/travel-blog-server<file_sep>/routes/articles.js
const express = require('express');
const router = express.Router();
const Articles = require('../models/articles');
const mongoose = require('mongoose');
var ObjectId = mongoose.Types.ObjectId;
// Get all Articles
router.get('/articles', (req, res) => {
if(req.query.id){
Articles.findById(req.query.id)
.then(article => res.json(article))
.catch(err => res.status(400).json(`Error: ${err}`))
}
else{
Articles.find()
.then(article => res.json(article))
.catch(err => res.status(400).json(`Error: ${err}`))
}
})
// create new Articles
router.post('/add', (req, res) => {
const newArticle = new Articles({
title: req.body.title,
article: req.body.article,
image: req.body.image,
authorname: req.body.authorname
})
newArticle
.save()
.then(() => res.json('The New Article posted Successfuly..!!!'))
.catch(err => res.status(400).json(err))
})
// get Article by id
router.get('/:id', (req, res) => {
console.log("req", req.query)
Articles.findById(req.query.id)
.then(article => res.json(article))
.catch(err => res.status(400).json(`Error: ${err}`))
})
// Update The Article by ID
router.put('/:id', (req, res) => {
console.log("Update Req", req.query.id)
Articles.findByIdAndUpdate(req.query.id).exec()
.then(article => {
article.title = req.body.title,
article.article = req.body.article,
article.image = req.body.image,
article.authorname = req.body.authorname
article
.save()
.then(() => res.json("Article Updated Successfully...!!"))
.catch(err => res.status(400).json(`Error: ${err}`))
})
.catch(err => res.status(400).json(`Error: ${err}`))
})
// Delete The Article By ID
router.delete('/:id', (req, res) => {
Articles.findByIdAndDelete(req.query.id).exec()
.then(() => res.json("Article is Deleted Successfuly"))
.catch(err => res.status(400).json(`Error: ${err}`))
}
)
module.exports = router | 5409cae893c33847fe7374cfa5fdbbbf04fe9e38 | [
"JavaScript"
] | 1 | JavaScript | Dia-Bhavsar/travel-blog-server | 204b2d4fb4e6612983f9ea6a9132b035b443e107 | 8df21a9192222833557cd31c9e01d4a2dd9a57d9 | |
refs/heads/master | <repo_name>morgyken/tutorsboardbizv1<file_sep>/app/Http/Controllers/AdminController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class AdminController extends Controller
{
/**
* Create a new controller instance.
*
* @return void
*/
public function __construct()
{
$this->middleware('auth:admin');
}
/**
* Show the application dashboard.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
return view('admin.home');
}
//admin post Quustion
//admin update Question
//Admin Mark Question as Complete
//admin Put Question on Revision
//Admin Dispute Question
//Admin Make Payments
}
| ca0a3d247884affeb8b0ca4da2b38365c29134d5 | [
"PHP"
] | 1 | PHP | morgyken/tutorsboardbizv1 | 162c10358be8d75aaae51ff02a0fdfafec5e0a07 | ae1795f7d975995358e9b0e1c0bf7408b3e3e201 | |
refs/heads/master | <repo_name>Timurgorez/iCraft<file_sep>/src/store/index.js
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
import product from './product/index'
import payment from './payment/index'
import shoppingBag from './shoppingBag/index'
export default new Vuex.Store({
modules: {
product: product,
payment: payment,
shoppingBag: shoppingBag,
}
})
<file_sep>/src/directives/index.js
import Vue from "vue";
Vue.directive("onMobileShow", {
bind(el) {
function modify() {
if (
/Android|webOS|iPhone|iPad|iPod|BlackBerry|IEMobile|Opera Mini/i.test(
navigator.userAgent
) ||
window.innerWidth < 992
) {
el.style.display = "flex";
} else {
el.style.display = "none";
}
}
modify();
window.addEventListener("resize", modify, { passive: true });
}
});
Vue.directive("onDesctopShow", {
bind(el) {
function modify() {
if (
/Android|webOS|iPhone|iPad|iPod|BlackBerry|IEMobile|Opera Mini/i.test(
navigator.userAgent
) ||
window.innerWidth < 992
) {
el.style.display = "none";
} else {
el.style.display = "flex";
}
}
modify();
window.addEventListener("resize", modify, { passive: true });
}
});
Vue.directive("splitTitle", {
bind(el) {
console.log(el.clientWidth);
function modify() {
// if(el.clientWidth > 0){
// const countOfSymbols = Math.floor((el.clientWidth - 20) / 20 * 2);
// if(el.innerText.length > countOfSymbols){
// el.innerText = el.innerText.substring(0, 53) + '...';
// }
// }
}
setTimeout(() => {modify();}, 0)
window.addEventListener("resize", modify, { passive: true });
}
});
<file_sep>/src/store/shoppingBag/index.js
export default {
state: {
productInBag: [],
selectedAddress: {},
shoppingType: {},
insurance: {},
shippingAdresses: [
{
id: 1,
name: "<NAME>",
country: "Ukraine",
state: "Kharkiv",
zip_code: "Ukraine",
city: "Kharkiv",
address: "per. Pushkina 12",
default: false
},
{
id: 2,
name: "<NAME>",
country: "Canada",
state: "Quebec",
zip_code: "134234",
city: "Ottawa",
address: "ave. Lenina 12",
default: false
},
{
id: 3,
name: "<NAME>",
country: "USA",
state: "Texas",
zip_code: "34523",
city: "Miniapalis",
address: "ave. Lenina 12",
default: false
}
]
},
mutations: {
addToBag(state, payload) {
state.productInBag.push(payload);
},
addToSelectedAddress(state, payload) {
state.selectedAddress[payload.sellerId] = payload.address.id;
},
initialiseUserBag(state) {
if (localStorage.getItem("userBag")) {
state.productInBag = JSON.parse(localStorage.getItem("userBag"));
}
if (localStorage.getItem("selectedAddress")) {
state.selectedAddress = JSON.parse(
localStorage.getItem("selectedAddress")
);
}
},
modifyFieldInBag(state, obj) {
state.productInBag.forEach(prodInBag => {
if (prodInBag.id == obj.id) {
prodInBag[obj.name] = obj.value;
}
});
localStorage.setItem("userBag", JSON.stringify(state.productInBag));
},
removeProductFromBag(state, id) {
console.log(id);
state.productInBag.forEach((prodInBag, index) => {
if (prodInBag.id == id) {
state.productInBag.splice(index, 1);
}
});
localStorage.setItem("userBag", JSON.stringify(state.productInBag));
},
modifyInsurance(state, { sellerId, value }) {
state.insurance[sellerId] = value;
},
modifyShoppingType(state, { sellerId, value }) {
state.shoppingType[sellerId] = value;
},
initialiseShoppingType(state) {
state.productInBag.forEach(el => {
state.shoppingType[el.sellerId] = null;
state.insurance[el.sellerId] = false;
});
},
modifyShippingAddress(state, obj) {
state.shippingAdresses.forEach(address => {
if (address.id == obj.newModel.id) {
address = obj.newModel;
}
});
if (state.selectedAddress) {
for (let sellerId in state.selectedAddress) {
console.log(sellerId);
if (state.selectedAddress[sellerId].id == obj.newModel.id) {
state.selectedAddress[sellerId] = obj.newModel;
}
}
}
},
addNewShippingAddress(state, address) {
address.id = (~~(Math.random() * 1e8)).toString(16);
state.shippingAdresses.push(address);
}
// addNewFieldToBag(state, id, fieldName, value){
// console.log(id, fieldName, value);
// state.productInBag.forEach((prodInBag) => {
// if (prodInBag.id == id) {
// prodInBag[fieldName] = value;
// }
// });
// localStorage.setItem("userBag", JSON.stringify(state.productInBag));
// }
},
actions: {
addProductToBag({ commit, state }, product) {
commit("addToBag", product);
localStorage.setItem("userBag", JSON.stringify(state.productInBag));
},
addAddressToSelectedAddress({ commit, state }, shipping) {
commit("addToSelectedAddress", shipping);
localStorage.setItem(
"selectedAddress",
JSON.stringify(state.selectedAddress)
);
}
},
getters: {
getProductsInBag(state) {
return state.productInBag;
},
countProductsInBag(state) {
return state.productInBag.length;
},
isSelectedAddress(state) {
return sellerId => {
return !!state.selectedAddress && !!state.selectedAddress[sellerId];
};
},
getSelectedAddress(state) {
return state.selectedAddress;
},
getSelectedAddressBySellerId(state) {
return sellerId => {
return state.shippingAdresses.find(address => {
return address.id == state.selectedAddress[sellerId];
});
};
},
getDefaultAddress(state) {
return state.shippingAdresses.find(address => {
return address.default;
});
},
getShippingAdresses(state) {
return state.shippingAdresses;
},
getInsurance(state) {
return state.insurance;
},
getShoppingType(state) {
return state.shoppingType;
},
getOneShippingAdresses(state) {
return addressId => {
return state.shippingAdresses.find(address => {
return address.id == addressId;
});
};
}
}
};
<file_sep>/src/router/index.js
import Vue from "vue";
import VueRouter from "vue-router";
import CollectionPage from "@/views/CollectionPage";
import ProductItem from "@/views/ProductItem";
import BagPage from "@/views/BagPage";
import CheckoutPage from "@/views/CheckoutPage";
import Purchase from "@/views/Purchase";
import CollectionPageNew from "@/views/CollectionPageNew";
import HomePage from "@/views/HomePage";
import Community from "@/views/Community";
Vue.use(VueRouter);
export default new VueRouter({
// mode: 'history',
base: process.env.BASE_URL,
routes: [
{
path: "/",
name: "HomePage",
props: true,
component: HomePage
},
{
path: "/new",
name: "CollectionPageNew",
component: CollectionPageNew
},
{
path: "/old",
name: "CollectionPage",
props: true,
component: CollectionPage
},
{
path: "/product/:id",
name: "ProductPage",
props: true,
component: ProductItem
},
{
path: "/shopping-bag",
name: "ShoppingBag",
component: BagPage
},
{
path: "/checkout",
name: "CheckoutPage",
component: CheckoutPage
},
{
path: "/purchase",
name: "Purchase",
component: Purchase
},
{
path: "/community",
name: "Community",
component: Community
}
],
scrollBehavior(to, from, savedPosition) {
if (savedPosition) {
return savedPosition;
} else {
return { x: 0, y: 0 };
}
}
});
<file_sep>/src/store/seller/index.js
export default {
state: {
sellers: [
{
id: "1",
name: "<NAME>",
description:
"I am a crochet and jewelry designer. | share my inner self with the world by creating things for people with love, care and attention to detail. My hope is for everyone to have at least one piece of unique handmade apparel that they can treasure, knowing it was made by someone who put their heart into it.",
location: {
country: "Russia",
city: "Moscow",
street: "per. Pushkina 12",
zip: "12456"
},
rating: {
count: 18,
stars: 4.56
}
},
{
id: "2",
name: "<NAME>",
description:
"I am a crochet and jewelry designer. | share my inner self with the world by creating things for people with love, care and attention to detail. My hope is for everyone to have at least one piece of unique handmade apparel that they can treasure, knowing it was made by someone who put their heart into it.",
location: {
country: "Canada",
city: "Ottava",
street: "per. Pushkina 12",
zip: "12456"
},
rating: {
count: 17,
stars: 1.83
}
},
{
id: "3",
name: "<NAME>",
description:
"I am a crochet and jewelry designer. | share my inner self with the world by creating things for people with love, care and attention to detail. My hope is for everyone to have at least one piece of unique handmade apparel that they can treasure, knowing it was made by someone who put their heart into it.",
location: {
country: "Ukraine",
city: "Kharkiv",
street: "per. Pushkina 12",
zip: "12456"
},
rating: {
count: 34,
stars: 2.34
}
},
{
id: "4",
name: "<NAME>",
description:
"I am a crochet and jewelry designer. | share my inner self with the world by creating things for people with love, care and attention to detail. My hope is for everyone to have at least one piece of unique handmade apparel that they can treasure, knowing it was made by someone who put their heart into it.",
location: {
country: "Italy",
city: "Milan",
street: "per. Pushkina 12",
zip: "12456"
},
rating: {
count: 9,
stars: 4.86
}
}
]
},
mutations: {
// addNewFieldToBag(state, id, fieldName, value){
// console.log(id, fieldName, value);
// state.productInBag.forEach((prodInBag) => {
// if (prodInBag.id == id) {
// prodInBag[fieldName] = value;
// }
// });
// localStorage.setItem("userBag", JSON.stringify(state.productInBag));
// }
},
actions: {
// addProductToBag({ commit, state }, product) {
// commit("addToBag", product);
// localStorage.setItem("userBag", JSON.stringify(state.productInBag));
// }
},
getters: {
getSellers(state) {
return state.sellers;
},
getSeller(state) {
return sellerId => {
return state.sellers.find(seller => {
return seller.id == sellerId;
});
};
}
}
};
<file_sep>/src/main.js
import Vue from "vue";
import App from "./App.vue";
import router from "./router";
import store from "./store";
import { BootstrapVue, IconsPlugin } from "bootstrap-vue";
import VueAwesomeSwiper from "vue-awesome-swiper";
import VueCardFormat from "vue-credit-card-validation";
import Notifications from "vue-notification";
import SwiperCore, { Autoplay, EffectFade } from 'swiper';
// Import Swiper styles
import 'swiper/swiper.scss';
import 'swiper/components/navigation/navigation.scss';
import 'swiper/components/pagination/pagination.scss';
import 'swiper/components/scrollbar/scrollbar.scss';
import "swiper/swiper-bundle.css";
// install Swiper components
SwiperCore.use([Autoplay, EffectFade]);
import "./directives/index.js";
Vue.use(BootstrapVue);
Vue.use(VueAwesomeSwiper);
Vue.use(IconsPlugin);
Vue.use(VueCardFormat);
Vue.use(Notifications);
// Import Bootstrap and Bootstrap-Vue css
import "bootstrap/dist/css/bootstrap.css";
import "bootstrap-vue/dist/bootstrap-vue.css";
Vue.config.productionTip = false;
new Vue({
router,
store,
beforeCreate() {
this.$store.commit("initialiseUserBag");
this.$store.commit("initialiseShoppingType");
},
render: h => h(App),
created() {
this.$store.dispatch("loadProducts");
}
}).$mount("#app");
| b20e3d7cdcd27ec832be55089e53ce9167dabd34 | [
"JavaScript"
] | 6 | JavaScript | Timurgorez/iCraft | 6fdaf6c1ab0f17abc6f9cc13112f27ebd111eb13 | 6440e3eeffb1043f8a4d283f317e0b4dfb272023 | |
refs/heads/master | <repo_name>dgc-nuna/bin<file_sep>/list_public_ips.py
import boto.ec2
import WH
if __name__ == '__main__':
wh = WH.WH()
wh.connect()
conn = boto.ec2.connect_to_region("us-west-2")
for a in conn.get_all_addresses():
print "%s" % (a.public_ip)
<file_sep>/test_WH.py
import WH
conn = WH.WH()
conn.connect()
r = conn.add_allowed_host(40466,"https","nunahealth.com")
print r.text
<file_sep>/report_wh_ec2_diffs.py
import WH
import boto.ec2
import json
import re
wh_site_id = 40466
if __name__ == '__main__':
wh = WH.WH()
wh.connect()
ahs = wh.get_allowed_hosts(wh_site_id)['allowed_hosts']
eps = wh.get_entry_points(wh_site_id)['entry_points']
sorted_ahs = [ h for h in sorted(ahs, key=lambda a: a['hostname'],) if re.search('\d+(\.\d+){3}',h['hostname'])]
conn = boto.ec2.connect_to_region("us-west-2")
ec2addrs = conn.get_all_addresses()
new = set([addr.public_ip for addr in ec2addrs if addr.public_ip])
old = set([addr['hostname'] for addr in sorted_ahs])
hosts_to_add = new - old
hosts_to_del = old - new
for addr in hosts_to_del:
print "Del %s" % addr
for addr in hosts_to_add:
print "Add %s" % addr
<file_sep>/WH.py
import yaml
import requests
import json
import os
import sys
import urllib
import pprint
api_root="https://sentinel.whitehatsec.com/api"
class WH:
def __init__(self):
with open(os.path.expanduser("~") + "/.wh","r") as ymlfile:
cfg = yaml.load(ymlfile)
self.password = cfg["<PASSWORD>"]
self.username = cfg["username"]
def connect(self):
self.session = requests.session()
r = self.session.post(
"%s/user/%s/login" % (api_root,urllib.quote(self.username)),
data={"password": self.password})
#sys.stderr.write(r.text + "\n")
return r
def add_allowed_host(self,site_id,scheme,host):
r = self.session.post(
"%s/site/%d/allowed_hosts" % (api_root,site_id),
data=json.dumps(
{
"scheme":scheme,
"hostname":host
}),
headers={"Content-Type":"application/json"}
)
print r.status_code
print r.text
return r
def add_entry_point(self,site_id,method,url):
r = self.session.post(
"%s/site/%d/entry_points" % (api_root,site_id),
data=json.dumps(
[{
"method": method,
"uri" : url
}]
),
headers={"Content-Type":"application/json"}
)
print r.status_code
print r.text
return r
def get_entry_points(self,site_id):
r = self.session.get(
"%s/site/%d/entry_points" % (api_root,site_id),
headers={"Accept":"application/json"}
)
return json.loads(r.text)
def get_allowed_hosts(self,site_id):
r = self.session.get(
"%s/site/%d/allowed_hosts" % (api_root,site_id),
headers={"Accept":"application/json"}
)
return json.loads(r.text)
def delete(self,href):
r = self.session.delete(
"%s/%s" % (api_root,href),
headers={"Accept":"application/json"}
)
return r
<file_sep>/apply_wh_ec2_diffs.py
import WH
import boto.ec2
import json
import re
wh_site_id = 40466
if __name__ == '__main__':
wh = WH.WH()
wh.connect()
ahs = wh.get_allowed_hosts(wh_site_id)['allowed_hosts']
eps = wh.get_entry_points(wh_site_id)['entry_points']
sorted_ahs = [ h for h in sorted(ahs, key=lambda a: a['hostname'],) if re.search('\d+(\.\d+){3}',h['hostname'])]
conn = boto.ec2.connect_to_region("us-west-2")
ec2addrs = conn.get_all_addresses()
new = set([addr.public_ip for addr in ec2addrs if addr.public_ip])
old = set([addr['hostname'] for addr in sorted_ahs])
hosts_to_add = new - old
hosts_to_del = old - new
for addr in hosts_to_del:
print "Del %s" % addr
for addr in hosts_to_add:
print "Add %s" % addr
eps_to_delete = [ep['href'] for ep in eps if ep['uri'] in set([ 'https://' + h for h in hosts_to_del]) | set([ 'http://' + h for h in hosts_to_del])]
ahs_to_delete = [ah['href'] for ah in ahs if ah['hostname'] in hosts_to_del]
for ep in eps_to_delete:
tail = ep[5:] # strip "/api/"
print "deleting %s" % tail
r = wh.delete(tail)
for ah in ahs_to_delete:
tail = ah[5:] # strip "/api/"
print "deleting %s" % tail
r = wh.delete(tail)
print r
for a in hosts_to_add:
print wh.add_allowed_host(wh_site_id,"http",a).request
print wh.add_allowed_host(wh_site_id,"https",a).request
print wh.add_entry_point(wh_site_id,"GET","http://" + a + "/").request
print wh.add_entry_point(wh_site_id,"GET","https://" + a + "/").request
<file_sep>/wh_add_public_ips.py
import boto.ec2
import WH
site_id=40466
if __name__ == '__main__':
wh = WH.WH()
wh.connect()
conn = boto.ec2.connect_to_region("us-west-2")
for a in conn.get_all_addresses():
print "%s" % (a.public_ip)
print wh.add_allowed_host(site_id,"http",a.public_ip).request
print wh.add_allowed_host(site_id,"https",a.public_ip).request
print wh.add_entry_point(site_id,"GET","http://" + a.public_ip + "/").request
print wh.add_entry_point(site_id,"GET","https://" + a.public_ip + "/").request
| 6d2b6a4e4f97e84f96516f7ec775571174ff8979 | [
"Python"
] | 6 | Python | dgc-nuna/bin | de0931db9eb18d50362dbda1d2019d8c2e889c30 | d620b806e99ddebaad1ea76ca57b5d17d8cfa0b5 | |
refs/heads/main | <repo_name>bege-swann/cyber-ROOM<file_sep>/dashboard.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>CyberRoom_Admin</title>
<link rel="stylesheet" href="dashboard.css">
<script src="javascript.js"></script>
</head>
<body>
<div class="header">
<div class="header_cadre">
<div class="cadre_logo">
</div>
<div class="cadre_admin">
<h2 class="title">𝗔𝗗𝗠𝗜𝗡</h2>
</div>
</div>
<div class="header_time">
<h2 class="in_time">
<p id="date"></p>
<p></p>
</h2>
</div>
</div>
<!-- Partie Création d'utilisateur-->
<div class="account">
<h2 class="account_titre">Ajouter un utilisateur</h2>
<form method="post">
<input type="text" class="account_first-name" placeholder="𝑬𝒏𝒕𝒓𝒆𝒛 𝒖𝒏 𝒏𝒐𝒎" name="nom">
<input type="text" class="account_last-name" placeholder="𝑬𝒏𝒕𝒓𝒆𝒛 𝒖𝒏 𝒑𝒓𝒆́𝒏𝒐𝒎" name="prenom">
<input type="submit" class="account_button" value="Valider">
</form>
</div>
<?php
if (isset($_POST['prenom']) & isset($_POST['nom'])){
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM clients');
$requete= 'INSERT INTO clients VALUES(NULL, "' . $_POST['nom'] . '", "' . $_POST['prenom'] . '")';
$resultat = $bdd -> query($requete);
if ($resultat)
echo "<p>Le contact a été ajouté</p>";
else
echo "<p>Erreur</p>";
$reponse->closeCursor();
}
?>
<!-- Partie Assignation de postes-->
<div class="reservations">
<h2 class="reservations_titre">Assigner un poste</h2>
<form method="post">
<input type="text" placeholder= "𝑬𝒏𝒕𝒓𝒆𝒛 𝒖𝒏 𝒏𝒐𝒎" name="nom">
<input type="text" placeholder= "𝑬𝒏𝒕𝒓𝒆𝒛 𝒖𝒏 𝒑𝒓𝒆́𝒏𝒐𝒎" name="prenom">
<input type="text" placeholder= "Choisissez un poste" name="poste">
<input type="text" placeholder="Choisissez un temps" name="temps">
<input type="submit" class="account_button" value="Valider">
</form>
</div>
<?php
if (isset($_POST['nom']) & isset($_POST['prenom']) & isset($_POST['poste'])){
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes');
$requete= 'UPDATE postes' ;
$resultat = $bdd -> query($requete);
}
?>
<!-- Partie information sur les postes-->
<div class="postes">
<div class="postes_1">
<div class="postes_img"></div>
<div class="postes_infos">
<ul class="poste_liste">
<li class="poste_titre">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=1');
while($donnees= $reponse->fetch()){
echo 'Poste n° ' . $donnees['numero'];
}
$reponse->closeCursor();
?>
</li>
<li class="poste_utilisateur">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=1');
while($donnees= $reponse->fetch()){
echo 'Utilisateur : ' . $donnees['utilisateur_nom'] . ' ' . $donnees['utlisateur_prenom'];
}
$reponse->closeCursor();
?>
</li>
<li class="poste_timer">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=1');
while($donnees= $reponse->fetch()){
echo 'Temps restant : ' . $donnees['temps_restant'];
}
$reponse->closeCursor();
?>
</li>
</ul>
</div>
</div>
<div class="postes_2">
<div class="postes_img"></div>
<div class="postes_infos">
<ul class="poste_liste">
<li class="poste_titre">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=2');
while($donnees= $reponse->fetch()){
echo 'Poste n° ' . $donnees['numero'];
}
$reponse->closeCursor();
?>
</li>
<li class="poste_utilisateur">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=2');
while($donnees= $reponse->fetch()){
echo 'Utilisateur : ' . $donnees['utilisateur_nom'] . ' ' . $donnees['utlisateur_prenom'];
}
$reponse->closeCursor();
?>
</li>
<li class="poste_timer">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=2');
while($donnees= $reponse->fetch()){
echo 'Temps restant : ' . $donnees['temps_restant'];
}
$reponse->closeCursor();
?>
</li>
</ul>
</div>
</div>
<div class="postes_3">
<div class="postes_img"></div>
<div class="postes_infos">
<ul class="poste_liste">
<li class="poste_titre">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=3');
while($donnees= $reponse->fetch()){
echo 'Poste n° ' . $donnees['numero'];
}
$reponse->closeCursor();
?>
</li>
<li class="poste_utilisateur">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=3');
while($donnees= $reponse->fetch()){
echo 'Utilisateur : ' . $donnees['utilisateur_nom'] . ' ' . $donnees['utlisateur_prenom'];
}
$reponse->closeCursor();
?>
</li>
<li class="poste_timer">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=3');
while($donnees= $reponse->fetch()){
echo 'Temps restant : ' . $donnees['temps_restant'];
}
$reponse->closeCursor();
?>
</li>
</ul>
</div>
</div>
<div class="postes_4">
<div class="postes_img"></div>
<div class="postes_infos">
<ul class="poste_liste">
<li class="poste_titre">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=4');
while($donnees= $reponse->fetch()){
echo 'Poste n° ' . $donnees['numero'];
}
$reponse->closeCursor();
?>
</li>
<li class="poste_utilisateur">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=4');
while($donnees= $reponse->fetch()){
echo 'Utilisateur : ' . $donnees['utilisateur_nom'] . ' ' . $donnees['utlisateur_prenom'];
}
$reponse->closeCursor();
?>
</li>
<li class="poste_timer">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=4');
while($donnees= $reponse->fetch()){
echo 'Temps restant : ' . $donnees['temps_restant'];
}
$reponse->closeCursor();
?>
</li>
</ul>
</div>
</div>
<div class="postes_5">
<div class="postes_img"></div>
<div class="postes_infos">
<ul class="poste_liste">
<li class="poste_titre">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=5');
while($donnees= $reponse->fetch()){
echo 'Poste n° ' . $donnees['numero'];
}
$reponse->closeCursor();
?>
</li>
<li class="poste_utilisateur">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=5');
while($donnees= $reponse->fetch()){
echo 'Utilisateur : ' . $donnees['utilisateur_nom'] . ' ' . $donnees['utlisateur_prenom'];
}
$reponse->closeCursor();
?>
</li>
<li class="poste_timer">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=5');
while($donnees= $reponse->fetch()){
echo 'Temps restant : ' . $donnees['temps_restant'];
}
$reponse->closeCursor();
?>
</li>
</ul>
</div>
</div>
<div class="postes_6">
<div class="postes_img"></div>
<div class="postes_infos">
<ul class="poste_liste">
<li class="poste_titre">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=6');
while($donnees= $reponse->fetch()){
echo 'Poste n° ' . $donnees['numero'];
}
$reponse->closeCursor();
?>
</li>
<li class="poste_utilisateur">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=6');
while($donnees= $reponse->fetch()){
echo 'Utilisateur : ' . $donnees['utilisateur_nom'] . ' ' . $donnees['utlisateur_prenom'];
}
$reponse->closeCursor();
?>
</li>
<li class="poste_timer">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=6');
while($donnees= $reponse->fetch()){
echo 'Temps restant : ' . $donnees['temps_restant'];
}
$reponse->closeCursor();
?>
</li>
</ul>
</div>
</div>
<div class="postes_7">
<div class="postes_img"></div>
<div class="postes_infos">
<ul class="poste_liste">
<li class="poste_titre">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=7');
while($donnees= $reponse->fetch()){
echo 'Poste n° ' . $donnees['numero'];
}
$reponse->closeCursor();
?>
</li>
<li class="poste_utilisateur">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=7');
while($donnees= $reponse->fetch()){
echo 'Utilisateur : ' . $donnees['utilisateur_nom'] . ' ' . $donnees['utlisateur_prenom'];
}
$reponse->closeCursor();
?>
</li>
<li class="poste_timer">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=7');
while($donnees= $reponse->fetch()){
echo 'Temps restant : ' . $donnees['temps_restant'];
}
$reponse->closeCursor();
?>
</li>
</ul>
</div>
</div>
<div class="postes_8">
<div class="postes_img"></div>
<div class="postes_infos">
<ul class="poste_liste">
<li class="poste_titre">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=8');
while($donnees= $reponse->fetch()){
echo 'Poste n° ' . $donnees['numero'];
}
$reponse->closeCursor();
?>
</li>
<li class="poste_utilisateur">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=8');
while($donnees= $reponse->fetch()){
echo 'Utilisateur : ' . $donnees['utilisateur_nom'] . ' ' . $donnees['utlisateur_prenom'];
}
$reponse->closeCursor();
?>
</li>
<li class="poste_timer">
<?php
$bdd = new PDO('mysql:host=127.0.0.1;dbname=cyber_room;charset=utf8', 'phpmyadmin', 'Workout974!',array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$reponse = $bdd->query('SELECT * FROM postes WHERE numero=8');
while($donnees= $reponse->fetch()){
echo 'Temps restant : ' . $donnees['temps_restant'];
}
$reponse->closeCursor();
?>
</li>
</ul>
</div>
</div>
</div>
<!-- Partie footer-->
<footer>
<div>
<ul class="mail">
<li><NAME><br> <a href="<EMAIL>" id="copy"><img src="images/email.png" width="20px"
height="20px" /></a>
<a href="https://www.linkedin.com/in/gaetan-lavit-d-hautefort-6231241b8/" target="blank"><img
src="images/linkedin-rond-300x300.png" width="20px" height="20px" /></a>
<a href="https://www.facebook.com/gaetan.lavit.5/" target="blank"><img
src="images/Facebook-share-icon.png" width="20px" height="20px" /></a>
</li>
<li><NAME>
<br> <a href="<EMAIL>" id="copy"><img src="images/email.png" width="20px"
height="20px" /></a>
<a href="https://www.linkedin.com/in/dimitri-gigan-80a52a184/" target="blank"><img
src="images/linkedin-rond-300x300.png" width="20px" height="20px" /></a>
<a href="https://www.facebook.com/dimitri.gigan" target="blank"><img
src="images/Facebook-share-icon.png" width="20px" height="20px" /></a>
</li>
<li>AH-KEN<NAME> <br> <a href="<EMAIL>" id="copy"><img src="images/email.png" width="20px"
height="20px" /></a>
<a href="https://www.linkedin.com/in/abel-ah-keng-4490891b8/" target="blank"><img
src="images/linkedin-rond-300x300.png" width="20px" height="20px" /></a>
<a href="https://www.facebook.com/abel.akn" target="blank"><img
src="images/Facebook-share-icon.png" width="20px" height="20px" /></a>
<li><NAME> <br> <a href="<EMAIL>" id="copy"><img src="images/email.png" width="20px"
height="20px" /></a>
<a href="https://www.linkedin.com/in/swann-bege-56702a1bb/" target="blank"><img
src="images/linkedin-rond-300x300.png" width="20px" height="20px" /></a>
<a href="https://www.facebook.com/wahib.Qlf/" target="blank"><img
src="images/Facebook-share-icon.png" width="20px" height="20px" /></a>
</ul>
</div>
<p class="copyright">© Copyright Cyber Room </p>
</footer>
</body>
</html>
| 019c0de77afeeb9eaa13af353acb65aa4c8f36a2 | [
"PHP"
] | 1 | PHP | bege-swann/cyber-ROOM | 9ab3fac55dfc0f840a0833ad7a852bab1d4806d7 | 08b6ac261d2344a1f876a4caef666b32263838df | |
refs/heads/master | <repo_name>jasonwiener/PostCycla<file_sep>/gpx/simplifyPosData.js
/*
Simplify.js, a high-performance JS polyline simplification library
mourner.github.io/simplify-js
Copyright (c) 2017, <NAME>
All rights reserved.
Redistribution and use in source and binary forms, with or without modification, are
permitted provided that the following conditions are met:
1. Redistributions of source code must retain the above copyright notice, this list of
conditions and the following disclaimer.
2. Redistributions in binary form must reproduce the above copyright notice, this list
of conditions and the following disclaimer in the documentation and/or other materials
provided with the distribution.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY
EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF
MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION)
HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR
TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
Changes:
Updated to fit PosData
*/
var xName = 'x';
var yName = 'y';
exports.SetXName = function(name)
{
xName = name;
}
exports.SetYName = function(name)
{
yName = name;
}
// to suit your point format, run search/replace for '[xName]' and '[yName]';
// for 3D version, see 3d branch (configurability would draw significant performance overhead)
// square distance between 2 points
function getSqDist(p1, p2) {
var dx = p1[xName] - p2[xName],
dy = p1[yName] - p2[yName];
return dx * dx + dy * dy;
}
// square distance from a point to a segment
function getSqSegDist(p, p1, p2) {
var x = p1[xName],
y = p1[yName],
dx = p2[xName] - x,
dy = p2[yName] - y;
if (dx !== 0 || dy !== 0) {
var t = ((p[xName] - x) * dx + (p[yName] - y) * dy) / (dx * dx + dy * dy);
if (t > 1) {
x = p2[xName];
y = p2[yName];
} else if (t > 0) {
x += dx * t;
y += dy * t;
}
}
dx = p[xName] - x;
dy = p[yName] - y;
return dx * dx + dy * dy;
}
// rest of the code doesn't care about point format
// basic distance-based simplification
function simplifyRadialDist(points, sqTolerance) {
var prevPoint = points[0],
newPoints = [prevPoint],
point;
for (var i = 1, len = points.length; i < len; i++) {
point = points[i];
if (getSqDist(point, prevPoint) > sqTolerance) {
newPoints.push(point);
prevPoint = point;
}
}
if (prevPoint !== point) newPoints.push(point);
return newPoints;
}
// simplification using optimized Douglas-Peucker algorithm with recursion elimination
function simplifyDouglasPeucker(points, sqTolerance) {
var len = points.length,
MarkerArray = typeof Uint8Array !== 'undefined' ? Uint8Array : Array,
markers = new MarkerArray(len),
first = 0,
last = len - 1,
stack = [],
newPoints = [],
i, maxSqDist, sqDist, index;
markers[first] = markers[last] = 1;
while (last) {
maxSqDist = 0;
for (i = first + 1; i < last; i++) {
sqDist = getSqSegDist(points[i], points[first], points[last]);
if (sqDist > maxSqDist) {
index = i;
maxSqDist = sqDist;
}
}
if (maxSqDist > sqTolerance) {
markers[index] = 1;
stack.push(first, index, index, last);
}
last = stack.pop();
first = stack.pop();
}
for (i = 0; i < len; i++) {
if (markers[i]) newPoints.push(points[i]);
}
return newPoints;
}
// both algorithms combined for awesome performance
exports.simplify = function(points, tolerance, highestQuality)
{
var sqTolerance = tolerance !== undefined ? tolerance * tolerance : 1;
points = highestQuality ? points : simplifyRadialDist(points, sqTolerance);
points = simplifyDouglasPeucker(points, sqTolerance);
return points;
}
<file_sep>/HW_Controller/mbed/main.cpp
/**
* PostCycla stm32 sensor and server controller
* Used to read a CNY 70 reflex sensor to sense rotational speed of the trainers roller
* and set the servo position (0..100%) to change the resistance
* (servo will be mounted to the trainer actuating the trainers bowden cable)
* Input:
* any character between 32...132 (0..100%) and newline as delimiter
*
* Output:
* (will be triggered every time an input was sent)
* Will send a JSON string containing:
* { "distance" : xxx, "speedms" : xxx, "servoPos" : xxx, "charSent" : xxx }
*
* distance => dinstance since the last time the sensor was read
* speedms => current speed in meters per second
* servoPos => current position of the servo setting the trainer resistence
* sentChar => the servo position can be set from 0...100% and will be sent to the STM as an character followed by a newline
* this sentChar was implemented for debugging and will show the last sent character (should be between 32 and 132)
*
* @author <NAME>
* @version 1.0
*/
#include "mbed.h"
#include "Servo.h"
Servo servo(D3); // servo on pin 3
Serial pc(USBTX, USBRX); // starting serial port for communication with PostCycla sensor module
DigitalOut ledOut(LED1); // previously used for debugging
InterruptIn lightBarrier(D12); // pin 12 will sence the voltage output of the CNY 70
Timeout distanceTimer;
Timer stopwatch;
float roller_circum = 0.1; // roller circumference in m
int rotation_Cnt = 0; // roller rotation counter
int timer_end = 0; // stopwatch timer end time
int timer_begin = 0; // stopwatch timer begin time
float distanceTotal = 0; // distance in m since last request
float speed = 0; // current speed in m/s
int charSent = 0;
float position = 0.5; // current servo position
float range = 0.0005; // current servo range
/*
ligth Barrier counter
Counts light barrier hits (rotations of roller)
Writes:
rotation_Cnt - adds one
*/
void lightBarriercallback()
{
rotation_Cnt++;
}
/*
Send request data
Send the requested data via serial output and resets total distance
*/
void sendRequestData()
{
pc.printf("{ \"distance\" : %.1f, \"speedms\" : %0.2f, \"servoPos\" : %.3f, \"charSent\" : %d }\n", distanceTotal, speed, position, charSent);
}
/*
distance timeout
Gets called every second and calculates distance and speed travelled.
Reads:
stopwatch - to get accurate time since last call (should be every second)
rotation_Cnt - rotations since last call of distance timeout
Writes:
distance - in m from rotation_Cnt and roller_circum
totalDistance - in m by addin distance
speed - in m/s calculated from stopwatch time and distance
rotation_Cnt - resets to 0 after read
timer_end - used for accurate timeing
timer_begin - used for accurate timeing
*/
void distanceTimeoutCallback()
{
// gets time passed since last distance update
timer_end = stopwatch.read_us();
int usPassed = timer_end - timer_begin;
float distance = (roller_circum * rotation_Cnt); // gets distance in m since last distanceTimer call
speed = (distance * usPassed) /1000000; // calcs speed in m/s from passed time and distance
distanceTotal += distance; // adds current distance to total distance
// sendRequestData();
// resets all for next timeout
rotation_Cnt = 0; // resets rotationCounter
distanceTimer.attach(&distanceTimeoutCallback,1); // restarts distanceTimer
stopwatch.start(); // restarts Stopwatch
timer_begin = stopwatch.read_us(); // stores last distance update time
}
/*
Set Servo
Sets requested servo postion
*/
void setServo(float pos)
{
position = pos;
servo.calibrate(range, 45.0);
servo = position;
}
int main()
{
// lightBarrier counter
lightBarrier.fall(&lightBarriercallback);
// checks Speed and distance ever second
distanceTimer.attach(&distanceTimeoutCallback,1);
stopwatch.start();
timer_begin = stopwatch.read_us();
int tmpChar = 0;
// checks input via searial for new request
while(1)
{
while(tmpChar != 10) // read till newLine
{
tmpChar = pc.getc();
if (tmpChar >= 32 && tmpChar <= 133) // only get valid chars and only the last one
{
charSent = tmpChar;
}
}
tmpChar = 0;
float newPos = ((float)(charSent - 32)) / 100.0;
setServo(newPos);
sendRequestData();
distanceTotal = 0; //always reset
}
}<file_sep>/test/hardware/testAntplusSensors.js
/**
* Ant+ Sensor test
* This test will try and get data from an Ant+ stick with connected heart rate and cadence sensors
* Should return a console ouput with the heart rate and cadence
*
* Example:
* { HeartRate: 95, Cadence : 80 }
*
* For further information see folder: "antplus"
* Will not give an error if one of the sensors is not connected/paired.. it will only return 0
*
* @author <NAME>
* @version 1.0
*/
var AntSensors = require('../../antplus/AntSensors.js');
var FgRed = "\x1b[31m";
var FgGreen = "\x1b[32m";
var readData = 10; // times to read data (reading once per second)
var showResults = function (data)
{
if (data.Success)
{
console.log(FgGreen);
} else
{
console.log(FgRed);
}
console.log(data);
console.log("\x1b[0m");
};
try
{
// sensor id's for my heartrate and cadence sensor
antSensors = new AntSensors(30250, 59056);
antSensors.Open();
var i = 0;
var interval = setInterval(function ()
{
console.log(antSensors.Data);
if (i > readData)
{
antSensors.Close();
clearInterval( interval);
}
i++;
}, 1000);
} catch(err)
{
showResults({Success: false, Data: err});
}<file_sep>/test/hardware/testSTMSensor.js
/**
* STM32 sensor test
* This test will try and get data from the STM32/Arduino and check the output
* Should return a console ouput with the data from the module
*
* Example:
* { "distance" : 0, "speedms" : 0, "servoPos" : 0, "sentChar" : 0 }
*
* For further information see folder: "stm"
*
* @author <NAME>
* @version 1.0
*/
var os = require('os');
var stm = require('../../stm/newstm.js');
var FgRed = "\x1b[31m";
var FgGreen = "\x1b[32m";
var readData = 10; // times to read data (reading once per second)
var i = 0;
var interval= null;
var getComPort = function()
{
if (os.platform() == "win32")
{
return "COM3"; // change this to your std com port
} else
{
return "/dev/ttyACM0"; // change this to your std com port
}
}
var showResults = function (data)
{
if (data.Success)
{
console.log(FgGreen);
} else
{
console.log(FgRed);
}
console.log(data);
console.log("\x1b[0m");
};
try
{
var stmSensors = new stm(getComPort());
stmSensors.on("SensorReady", function (data)
{
console.log("Sensor Ready... sending data");
setTimeout(function()
{
interval = setInterval(function ()
{
stmSensors.SetLevel((i/10), false);
if (i > readData)
{
stmSensors.Disconnect();
clearInterval(interval);
}
i++;
}, 1000);
},3000); // need to wait at least 2 sec. ?!?! otherwise it wont work
});
stmSensors.on("SensorData", function (data)
{
// expected data = { distance: 0.1, speedms: 0, servoPos: 0.1 }
console.log(data);
});
stmSensors.Connect();
} catch(err)
{
showResults({Success: false, Data: err});
}
<file_sep>/stm/stmMock.js
/**
* STM32 sensor mockup
* This mockup will provide sensor data for testing
* Constant speed can be set with the testMS variable (meters per second).. currently set to 24 km/h
* Should provide the same data as the STM32 hardware
* Example:
* { "distance" : 0, "speedms" : 0, "servoPos" : 0, "sentChar" : 0 }
*
* distance => dinstance since the last time the sensor was read
* speedms => current speed in meters per second
* servoPos => current position of the servo setting the trainer resistence
* sentChar => the servo position can be set from 0...100% and will be sent to the STM as an character followed by a newline
* this sentChar was implemented for debugging and will show the last sent character (should be between 32 and 132)
*
* For more details regarding the functionality of this module look at the "newstm.js" containing the real implementation.
* @author <NAME>
* @version 1.0
*/
var events = require('events');
var Settings = require('../settings.js');
var calc = require('../util/calc.js');
var testMS = 6.666;
var ServoOffset = { Min : 0.2, Max: 0.9};
var STM = function(comPort, script, tcpPort, ip)
{
events.EventEmitter.call(this);
this.ServerPos = 0;
this.SensorReady = function()
{
this.emit('SensorReady');
}
this.SensorData = function(strdata)
{
var data = JSON.parse(strdata);
this.emit('SensorData', data);
}
}
STM.prototype.__proto__ = events.EventEmitter.prototype;
STM.prototype.Connect = function()
{
this.Init();
}
STM.prototype.Disconnect = function()
{
}
STM.prototype.Destroy = function()
{
}
STM.prototype.Init = function()
{
ServoOffset = typeof Settings.Data.Sensors.ServoOffset !== 'undefined' ? Settings.Data.Sensors.ServoOffset : ServoOffset;
ServoOffset.Delta = ServoOffset.Max - ServoOffset.Min;
var me = this;
setTimeout(function(){
me.SensorReady();
},1000);
}
STM.prototype.SetLevel = function(pos)
{
// servo controller expects values from 32 to 132
pos = checkServoEndpoints(pos);
var position = pos;
pos = (pos *100) +32;
var send = String.fromCharCode(pos);
if (Settings.Data.DebugOutput)
{
console.log("Char: %s Pos: %d CHR: %d", send, position, send.charCodeAt(0));
}
this.SensorData("{ \"distance\" : "+testMS+", \"speedms\" : "+testMS+", \"servoPos\" : "+pos+", \"sentChar\" : "+send.charCodeAt(0)+" }");
}
// determined by enpoints of trainer bowden cable
var checkServoEndpoints = function(pos)
{
var offestPos = calc.ceil(pos /1 * ServoOffset.Delta);
offestPos += ServoOffset.Min;
if (offestPos > ServoOffset.Max)
{
offestPos = ServoOffset.Max;
}
if (offestPos < ServoOffset.Min)
{
offestPos = ServoOffset.Min;
}
return offestPos;
}
// export the class
module.exports = STM;
<file_sep>/test/hardware/libs/test_antplus.js
var Ant = require('../../../antplus/antplus.js');
var events = require('events');
var resultData = {
Test: 'AntPlus',
Success: false,
Data: null};
var Test = function()
{
events.EventEmitter.call(this);
var me = this;
this.Result = function(success, data)
{
resultData.Success = success;
resultData.Data = data;
this.emit('TestResult', resultData);
}
this.Setup = function(data)
{
console.log('AntPlus setup');
try
{
me.stick = new Ant.GarminStick2();
me.stick.on('startup', function ()
{
console.log('Ant stick started');
try
{
me.stick.detach_all();
me.stick.close();
} catch(err)
{
me.Result(false,err);
}
});
me.stick.on('shutdown', function ()
{
me.Result(true, 'Ant Stick started and shut down gracefully!');
});
} catch(err)
{
me.Result(false,err);
}
}
this.Test = function()
{
try
{
console.log("checking ant plus stick");
if (!me.stick.open())
{
me.Result(false, 'Stick not Found');
return;
}
//me.Result(true, 'Everything fine');
} catch(err)
{
me.Result(false,err);
}
}
}
Test.prototype.__proto__ = events.EventEmitter.prototype;
// export the class
module.exports = Test;<file_sep>/antplus/AntSensorsMock.js
/**
* Ant+ sensors (heart rate and cadence) mockup
* This mockup will provide sensor data for testing
* Constant cadence and heart rate can be set in the "AntSensors" function
* Should provide the same data as the "AntSensors.js"
* Example:
* { HeartRate: 95, Cadence : 80 }
**
* For more details regarding the functionality of this module look at the "AntSensors.js" containing the real implementation.
* @author <NAME>
* @version 1.0
*/
var Settings = require('../settings.js');
var Ant = require('./antplus.js');
var AntSensors = function(HR_dev_id, CAD_dev_id)
{
this.Data = { HeartRate: 95, Cadence : 80 };
}
AntSensors.prototype.Open = function()
{
}
AntSensors.prototype.Close = function()
{
}
module.exports = AntSensors;
<file_sep>/test/hardware/libs/test_usb.js
var usbPort = require('usb');
var events = require('events');
var resultData = {
Test: 'USBPort',
Success: false,
Data: null};
var Test = function()
{
events.EventEmitter.call(this);
var me = this;
this.Result = function(success, data)
{
resultData.Success = success;
resultData.Data = data;
this.emit('TestResult', resultData);
}
this.Setup = function(data)
{
console.log('USB setup');
try
{
} catch(err)
{
me.Result(false,err);
}
}
this.Test = function()
{
try
{
console.log("checking usb devices");
var list = usbPort.getDeviceList();
me.Result(true, list);
} catch(err)
{
me.Result(false,err);
}
}
}
Test.prototype.__proto__ = events.EventEmitter.prototype;
// export the class
module.exports = Test;<file_sep>/antplus/antplus.js
/**
* Ant+ sensors (heart rate and cadence)
* This encapsulates the sensors to a single module
* The implementation was converted from TypeScript provided by:
* <NAME> https://github.com/Loghorn/ant-plus
*
* @author <NAME>
* @version 1.0
*/
var Ant = require('./ant.js');
//*
var HRS = require('./HeartRateSensor.js');
var CS = require('./CadenceSensor.js');
//*/
module.exports = {
GarminStick2: Ant.GarminStick2,
GarminStick3: Ant.GarminStick3,
//*
HeartRateSensor: HRS.HeartRateSensor,
HeartRateScanner: HRS.HeartRateScanner,
CadenceSensor: CS.CadenceSensor,
CadenceScanner: CS.CadenceScanner
//*/
};<file_sep>/antplus/HeartRateSensor.js
var events = require("events");
var Ant = require("./ant.js");
var __extends = (this && this.__extends) || function (d, b) {
for (var p in b) if (b.hasOwnProperty(p)) d[p] = b[p];
function __() { this.constructor = d; }
d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());
};
var Constants = Ant.Constants;
var Messages = Ant.Messages;
var HeartRateSensorState = (function () {
function HeartRateSensorState(deviceId) {
this.DeviceID = deviceId;
}
return HeartRateSensorState;
}());
var HeartRateScannerState = (function (_super) {
__extends(HeartRateScannerState, _super);
function HeartRateScannerState() {
_super.apply(this, arguments);
}
return HeartRateScannerState;
}(HeartRateSensorState));
var PageState;
(function (PageState) {
PageState[PageState["INIT_PAGE"] = 0] = "INIT_PAGE";
PageState[PageState["STD_PAGE"] = 1] = "STD_PAGE";
PageState[PageState["EXT_PAGE"] = 2] = "EXT_PAGE";
})(PageState || (PageState = {}));
var HeartRateSensor = (function (_super) {
__extends(HeartRateSensor, _super);
function HeartRateSensor(stick) {
_super.call(this, stick);
this.pageState = PageState.INIT_PAGE; // sets the state of the receiver - INIT, STD_PAGE, EXT_PAGE
this.decodeDataCbk = this.decodeData.bind(this);
}
HeartRateSensor.prototype.attach = function (channel, deviceID) {
_super.prototype.attach.call(this, channel, 'receive', deviceID, HeartRateSensor.deviceType, 0, 255, 8070);
this.state = new HeartRateSensorState(deviceID);
};
HeartRateSensor.prototype.decodeData = function (data) {
if (data.readUInt8(Messages.BUFFER_INDEX_CHANNEL_NUM) !== this.channel) {
return;
}
switch (data.readUInt8(Messages.BUFFER_INDEX_MSG_TYPE)) {
case Constants.MESSAGE_CHANNEL_BROADCAST_DATA:
case Constants.MESSAGE_CHANNEL_ACKNOWLEDGED_DATA:
case Constants.MESSAGE_CHANNEL_BURST_DATA:
if (this.deviceID === 0) {
this.write(Messages.requestMessage(this.channel, Constants.MESSAGE_CHANNEL_ID));
}
var page = data.readUInt8(Messages.BUFFER_INDEX_MSG_DATA);
if (this.pageState === PageState.INIT_PAGE) {
this.pageState = PageState.STD_PAGE; // change the state to STD_PAGE and allow the checking of old and new pages
}
else if ((page !== this.oldPage) || (this.pageState === PageState.EXT_PAGE)) {
this.pageState = PageState.EXT_PAGE; // set the state to use the extended page format
switch (page & ~HeartRateSensor.TOGGLE_MASK) {
case 1:
//decode the cumulative operating time
this.state.OperatingTime = data.readUInt8(Messages.BUFFER_INDEX_MSG_DATA + 1);
this.state.OperatingTime |= data.readUInt8(Messages.BUFFER_INDEX_MSG_DATA + 2) << 8;
this.state.OperatingTime |= data.readUInt8(Messages.BUFFER_INDEX_MSG_DATA + 3) << 16;
this.state.OperatingTime *= 2;
break;
case 2:
//decode the Manufacturer ID
this.state.ManId = data.readUInt8(Messages.BUFFER_INDEX_MSG_DATA + 1);
//decode the 4 byte serial number
this.state.SerialNumber = this.deviceID;
this.state.SerialNumber |= data.readUInt16LE(Messages.BUFFER_INDEX_MSG_DATA + 2) << 16;
this.state.SerialNumber >>>= 0;
break;
case 3:
//decode HW version, SW version, and model number
this.state.HwVersion = data.readUInt8(Messages.BUFFER_INDEX_MSG_DATA + 1);
this.state.SwVersion = data.readUInt8(Messages.BUFFER_INDEX_MSG_DATA + 2);
this.state.ModelNum = data.readUInt8(Messages.BUFFER_INDEX_MSG_DATA + 3);
break;
case 4:
//decode the previous heart beat measurement time
this.state.PreviousBeat = data.readUInt16LE(Messages.BUFFER_INDEX_MSG_DATA + 2);
break;
default:
break;
}
}
// decode the last four bytes of the HRM format, the first byte of this message is the channel number
this.DecodeDefaultHRM(data.slice(Messages.BUFFER_INDEX_MSG_DATA + 4));
this.oldPage = page;
break;
case Constants.MESSAGE_CHANNEL_ID:
this.deviceID = data.readUInt16LE(Messages.BUFFER_INDEX_MSG_DATA);
this.transmissionType = data.readUInt8(Messages.BUFFER_INDEX_MSG_DATA + 3);
this.state.DeviceID = this.deviceID;
break;
default:
break;
}
};
HeartRateSensor.prototype.DecodeDefaultHRM = function (pucPayload) {
// decode the measurement time data (two bytes)
this.state.BeatTime = pucPayload.readUInt16LE(0);
// decode the measurement count data
this.state.BeatCount = pucPayload.readUInt8(2);
// decode the measurement count data
this.state.ComputedHeartRate = pucPayload.readUInt8(3);
this.emit('hbdata', this.state);
};
HeartRateSensor.deviceType = 120;
HeartRateSensor.TOGGLE_MASK = 0x80;
return HeartRateSensor;
}(Ant.AntPlusSensor));
exports.HeartRateSensor = HeartRateSensor;
var HeartRateScanner = (function (_super) {
__extends(HeartRateScanner, _super);
function HeartRateScanner(stick) {
_super.call(this, stick);
this.states = {};
this.pageState = PageState.INIT_PAGE;
this.decodeDataCbk = this.decodeData.bind(this);
}
HeartRateScanner.prototype.scan = function () {
_super.prototype.scan.call(this, 'receive');
};
HeartRateScanner.prototype.decodeData = function (data) {
if (data.length <= Messages.BUFFER_INDEX_EXT_MSG_BEGIN || !(data.readUInt8(Messages.BUFFER_INDEX_EXT_MSG_BEGIN) & 0x80)) {
console.log('wrong message format');
return;
}
var deviceId = data.readUInt16LE(Messages.BUFFER_INDEX_EXT_MSG_BEGIN + 1);
var deviceType = data.readUInt8(Messages.BUFFER_INDEX_EXT_MSG_BEGIN + 3);
if (deviceType !== HeartRateScanner.deviceType) {
return;
}
if (!this.states[deviceId]) {
this.states[deviceId] = new HeartRateScannerState(deviceId);
}
if (data.readUInt8(Messages.BUFFER_INDEX_EXT_MSG_BEGIN) & 0x40) {
if (data.readUInt8(Messages.BUFFER_INDEX_EXT_MSG_BEGIN + 5) === 0x20) {
this.states[deviceId].Rssi = data.readInt8(Messages.BUFFER_INDEX_EXT_MSG_BEGIN + 6);
this.states[deviceId].Threshold = data.readInt8(Messages.BUFFER_INDEX_EXT_MSG_BEGIN + 7);
}
}
switch (data.readUInt8(Messages.BUFFER_INDEX_MSG_TYPE)) {
case Constants.MESSAGE_CHANNEL_BROADCAST_DATA:
case Constants.MESSAGE_CHANNEL_ACKNOWLEDGED_DATA:
case Constants.MESSAGE_CHANNEL_BURST_DATA:
var page = data.readUInt8(Messages.BUFFER_INDEX_MSG_DATA);
if (this.pageState === PageState.INIT_PAGE) {
this.pageState = PageState.STD_PAGE; // change the state to STD_PAGE and allow the checking of old and new pages
}
else if ((page !== this.oldPage) || (this.pageState === PageState.EXT_PAGE)) {
this.pageState = PageState.EXT_PAGE; // set the state to use the extended page format
switch (page & ~HeartRateScanner.TOGGLE_MASK) {
case 1:
//decode the cumulative operating time
this.states[deviceId].OperatingTime = data.readUInt8(Messages.BUFFER_INDEX_MSG_DATA + 1);
this.states[deviceId].OperatingTime |= data.readUInt8(Messages.BUFFER_INDEX_MSG_DATA + 2) << 8;
this.states[deviceId].OperatingTime |= data.readUInt8(Messages.BUFFER_INDEX_MSG_DATA + 3) << 16;
this.states[deviceId].OperatingTime *= 2;
break;
case 2:
//decode the Manufacturer ID
this.states[deviceId].ManId = data.readUInt8(Messages.BUFFER_INDEX_MSG_DATA + 1);
//decode the 4 byte serial number
this.states[deviceId].SerialNumber = this.deviceID;
this.states[deviceId].SerialNumber |= data.readUInt16LE(Messages.BUFFER_INDEX_MSG_DATA + 2) << 16;
this.states[deviceId].SerialNumber >>>= 0;
break;
case 3:
//decode HW version, SW version, and model number
this.states[deviceId].HwVersion = data.readUInt8(Messages.BUFFER_INDEX_MSG_DATA + 1);
this.states[deviceId].SwVersion = data.readUInt8(Messages.BUFFER_INDEX_MSG_DATA + 2);
this.states[deviceId].ModelNum = data.readUInt8(Messages.BUFFER_INDEX_MSG_DATA + 3);
break;
case 4:
//decode the previous heart beat measurement time
this.states[deviceId].PreviousBeat = data.readUInt16LE(Messages.BUFFER_INDEX_MSG_DATA + 2);
break;
default:
break;
}
}
// decode the last four bytes of the HRM format, the first byte of this message is the channel number
this.DecodeDefaultHRM(deviceId, data.slice(Messages.BUFFER_INDEX_MSG_DATA + 4));
this.oldPage = page;
break;
default:
break;
}
};
HeartRateScanner.prototype.DecodeDefaultHRM = function (deviceId, pucPayload) {
// decode the measurement time data (two bytes)
this.states[deviceId].BeatTime = pucPayload.readUInt16LE(0);
// decode the measurement count data
this.states[deviceId].BeatCount = pucPayload.readUInt8(2);
// decode the measurement count data
this.states[deviceId].ComputedHeartRate = pucPayload.readUInt8(3);
this.emit('hbdata', this.states[deviceId]);
};
HeartRateScanner.deviceType = 120;
HeartRateScanner.TOGGLE_MASK = 0x80;
return HeartRateScanner;
}(Ant.AntPlusScanner));
exports.HeartRateScanner = HeartRateScanner;
<file_sep>/test/calculations/TrackParsingAndSmoothing_test.js
/**
* GPX track parsing and smoothing
* Tests the gpx file parser and smoothing algorithm
* Needs the original example/testing track gpx file (track segment length is fixed for testing)
* Should result in a console output either showing an error or the new smoothed segments
*
* @author <NAME>
* @version 1.0
*/
var Settings = require('../../settings.js');
var parseString = require('xml2js').parseString;
var gpxParser = require('../../gpx/parseGPX.js');
var files = require('../../files.js');
var readGPX = function (fileContent)
{
parseString(fileContent, function (err, result)
{
var segments = gpxParser.parse(result.gpx.trk[0].trkseg[0].trkpt);
if (segments.length >= 1003) // original length of Example/Testing track
{
console.log("Error track segments not smoothed.");
return;
}
segments.forEach(function(element)
{
if (element)
{
console.log(element);
} else
{
console.log("Error: ", element);
}
}, this);
});
}
files.ReadFileContent({ file : "Example_Testing Track.gpx"}, readGPX);<file_sep>/postcycla.js
/**
* PostCycla
* Will create a web server/sockets to provide & handle client (html) requests
*
* @author <NAME>
* @version 1.0
*/
var Settings = require('./settings.js'); // this "First"
var app = require('express')();
var http = require('http').Server(app);
var path = require('path');
var drive = require('./drive.js');
var files = require('./files.js');
var States = require("./serviceState.js");
var io = require('socket.io')(http);
drive.Init();
// setting web server path for client files
app.use('/', require('express').static(path.join(__dirname, 'newclient')));
//*** WebSocket request handling *//*
States.SetStateCallback(function(state)
{
io.local.emit("GetState", state);
})
// receiving from client
io.on('connection', function(socket)
{
socket.on('CancelRide', function(data)
{
drive.CancelRide();
});
socket.on("StartRide", function(data)
{
drive.StartRide();
});
socket.on("StopRide", function(data)
{
drive.StopRide()
});
socket.on("GetState", function(data)
{
io.local.emit("GetState", States.GetCurrentState());
});
socket.on("GetFiles", function()
{
files.GetFiles(function(data, err)
{
if (err)
{
io.local.emit("Error", err);
} else
{
io.local.emit("GetFiles", data);
}
});
});
socket.on("GetMountain", function()
{
io.local.emit("GetMountain", drive.GetMountain());
});
socket.on("LoadTrack",function(data)
{
files.ReadFileContent(data, drive.readGPX); // call file Read and parse callback
});
socket.on("GetOptions", function()
{
io.local.emit("GetOptions", Settings.Data);
});
socket.on("SaveOptions", function(req, resp)
{
try
{
if (req)
{
Settings.Data.BikeWeightKG = req.BikeWeightKG;
Settings.Data.MinimumJoulperMeter = req.MinimumJoulperMeter;
Settings.Data.RiderWeightKG = req.RiderWeightKG;
Settings.Data.Smooth = req.Smooth;
Settings.Save();
io.local.emit("SaveOptions", "OK");
}
}
catch(e)
{
console.log("Error save Options:",e)
}
});
socket.on("GetPowerSettings", function()
{
io.local.emit("GetPowerSettings", Settings.Data.PowerCalc);
});
socket.on("UpdatePowerSettings", function(req, resp)
{
try
{
if (req)
{
Settings.Data.PowerCalc.FrontArea = req.FrontArea;
Settings.Data.PowerCalc.DragCoeff = req.DragCoeff;
Settings.Data.PowerCalc.DriveDrainLoss = req.DriveDrainLoss;
Settings.Data.PowerCalc.RollingResistance = req.RollingResistance;
Settings.Data.PowerCalc.PowerOffset = req.PowerOffset;
Settings.Save();
io.local.emit("UpdatePowerSettings", "OK");
}
}
catch(e)
{
console.log("Error save Options:",e)
}
});
if (Settings.Data.DebugOutput)
{
console.log('a user connected');
}
});
drive.SetPosUpdateCallback(function(PosData)
{
io.local.emit("PosUpdate", PosData);
});
// WebSocket handling */
//*** WebServer request handling (REST) *//*
app.get("/GetState", function(req, resp)
{
resp.send(States.GetCurrentState());
});
app.get("/LoadFileList", function(req, resp)
{
resp.send(files.LoadFileList());
});
app.get("/GetFileList", function(req, resp)
{
resp.send(files.GetFileList());
});
app.get("/LoadTrack", function(req, resp)
{
resp.send(files.ReadFileContent(req.query, drive.readGPX)); // call file Read and parse callback
});
app.get("/GetOptions", function(req, resp)
{
resp.send(Settings.Data);
});
app.get("/SaveOptions", function(req, resp)
{
if (req.query)
{
Settings.Data.BikeWeightKG = req.query.BikeWeightKG;
Settings.Data.MinimumJoulperMeter = req.query.MinimumJoulperMeter;
Settings.Data.RiderWeightKG = req.query.RiderWeightKG;
Settings.Data.Smooth = req.query.Smooth;
Settings.Save();
}
resp.send("OK");
});
app.get("/GetSettings", function(req, resp)
{
resp.send(Settings.Data);
});
app.get("/CancelRide", function(req, resp)
{
resp.send(drive.CancelRide());
});
app.get("/StartRide", function(req, resp)
{
resp.send(drive.StartRide());
});
app.get("/StopRide", function(req, resp)
{
resp.send(drive.StopRide());
});
app.get("/GetPos", function(req, resp)
{
resp.send(drive.GetPositionData());
});
app.get("/GetMountain", function(req, resp)
{
resp.send(drive.GetMountain());
});
// REST handling */
http.listen(Settings.Data.HttpPort);
console.log('Server running at http://localhost:'+Settings.Data.HttpPort+'/');
<file_sep>/settings.js
/**
* Settings module
* Will automatically load settings stored inside the settings.json file
*
* Settings json Example:
*
*{
* "UseMockups": true, => if "true" PostCycla will run without the need of sensors (FOR TESTING)
* "DebugOutput": false, => will show more information during run in the console output
* "PowerCalc":
* {
* "MinimumJoulperMeter": 16, => minimum joul/watt per meter (for going downhill....)
* "BikeWeightKG" : 10, => weight of bike and equipment (needed for power calculation)
* "RiderWeightKG" : 95, => weight of yourself (needed for power calculation)
* "FrontArea" : 0.55, => frontal area, rider+bike (m^2) (original 0,509)
* "DragCoeff" : 0.7, => drag coefficient Cd (original 0,63)
* "DriveDrainLoss" : 4, => drivetrain loss Loss_dt (original 3%)
* "RollingResistance" : 0.005, => coefficient of rolling resistance Crr
* "PowerOffset" : 1.1 => used to calculate power offset for fine tuning 1 = 100% (used in PowerCalcPostCycla.js)
* }
* "WebSocketPort" : 9998, => port for the web socket (needed for client communication)
* "HttpPort" : 8125, => port for the web server (needed for the client )
* "GPXDir": "./tracks", => path to the directory where gpx files will be read and written
* "Smooth": 0.2, => parameter for gpx parsing and smoothing algorithm
*
* "Sensors":
* {
* "HeartRate_AntId" : 30250, => heart rate sensor Ant+ id (needed for Ant+ and pairing)
* "Cadence_AntId": 59056, => cadence sensor Ant+ id (needed for Ant+ and pairing)
* "STM":
* {
* "ComPort" : "/dev/ttyACM0", => serial port for connection with the STM sensor
* "ServoOffset": => offset for min/max travel of the servo (will normally go from 0..1)
* {
* "Min" : 0.2,
* "Max" : 0.9
* }
* }
* }
*}
*
* For further information about this data check the related modules.
*
* @author <NAME>
* @version 1.0
*/
var fs = require('fs');
var settingsfile = "./settings.json";
/**
* Data
* data stored inside the settingsfile
*/
exports.Data = null;
/**
* Load
* Loading settings form the "settingsfile"
*/
exports.Load = function()
{
exports.Data = JSON.parse(fs.readFileSync(settingsfile).toString());
}
/**
* Rest
* Reload/Reset settings form the "settingsfile"
*/
exports.Reset = function()
{
exports.Data = JSON.parse(fs.readFileSync(settingsfile).toString());
}
/**
* Save
* Save settings to the "settingsfile"
*/
exports.Save = function()
{
try
{
fs.writefileSync(settingsfile, JSON.stringify(exports.Data));
} catch(e)
{}
}
exports.Load(); // Load settings automatically<file_sep>/util/calc.js
// Math ceiling function with the default for of 2 digits
exports.ceil = function(f, digits)
{
digits = typeof digits !== 'undefined' ? digits : 2;
var div = Math.pow(10 ,digits);
if (f > 0)
{
f = Math.ceil(f * div) /div;
} else
{
f = Math.floor(f *div) /div;
}
return parseFloat(f.toFixed(digits));
}
// Math floor function with default the set for 2 digits
exports.floor = function(f, digits)
{
digits = typeof digits !== 'undefined' ? digits : 2;
var div = Math.pow(10 ,digits);
if (f > 0)
{
f = Math.floor(f *div) /div;
} else
{
f = Math.ceil(f *div) /div;
}
return parseFloat(f.toFixed(digits));
}<file_sep>/drive.js
/**
* Drive module
* Reads and writes GPX data
* Loads the GPX file and uses sensor data to calculate
* - Position
* - Power/Resistance
* - Data for UI & file output
*
* @author <NAME>
* @version 1.0
*/
// --- required modules
var fs = require('fs');
var path = require('path');
var parseString = require('xml2js').parseString;
var calc = require('./util/calc.js');
var sensors = require('./sensors.js');
var gpxWriter = require('./gpx/GPXWriter.js');
var gpxParser = require('./gpx/parseGPX.js');
var latlon = require('./gps/latlon.js');
var Settings = require('./settings.js');
var PowerCalculator = require('./power/PowerCalcPostCycla.js'); // Implement equipment calculations here
var States = require("./serviceState.js");
// --- Fields
var sensorTimer;
var PowerCalc;
var Data =
{
elediv: 0,
recordCnt: 0,
segments: new Array(),
odo : 0,
lastSegIdx : 0,
lastWrittenSeg : 0,
writeDir: "",
}
var PosData =
{
pos:
{
lat: 0,
lng: 0,
slope: 0,
ele: 0
},
total: 0,
power :
{
watt: 0,
resPerc: 0,
resistance: 0
},
odo: 0,
starttime: 0,
time: 0,
bike:
{
speed: 0,
cad: 0,
pulse: 0
}
};
// --- exports
exports.State
var PosUpdateCallback = null;
/**
* Initialization
* Starts PowerCalculator & sets output directory form the saved settings
*/
exports.Init = function()
{
PowerCalc = new PowerCalculator();
Data.writeDir = Settings.Data.GPXDir;
}
exports.SetPosUpdateCallback = function(callback)
{
PosUpdateCallback = callback;
}
/**
* readGPX
* parses the gpx file content and starts sensors for ride
*/
exports.readGPX = function (fileContent)
{
Data.odo = 0;
Data.lastSegIdx = 0;
Data.lastWrittenSeg = 0;
parseString(fileContent, function (err, result)
{
Data.segments = gpxParser.parse(result.gpx.trk[0].trkseg[0].trkpt);
States.SetCurrentState(States.States.TrackLoaded);
States.SetCurrentState(States.States.StartingSensors);
sensors.SetSensorDataCallback(sensorReader);
sensors.Start(function()
{
States.SetCurrentState(States.States.ReadyForRide);
});
});
}
/**
* GetPositionData
* returns the current position and all data for it
*/
exports.GetPositionData = function()
{
return PosData;
}
/**
* StartRide
* sets the state accordingly
* creates a new gpx file
* sets start time
* creates a timer to update ride calculations every second
*/
exports.StartRide = function()
{
States.SetCurrentState(States.States.RecordingTrack);
gpxWriter.NewFile(Data.writeDir);
PosData.starttime = (new Date()).getTime();
sensorTimer = setInterval(function ()
{
rideinterval();
}, 1000);
return "OK";
}
/**
* StopRide
* stops all sensors
* closes gpx file
* stops ride calculation timer
* updates state
*/
exports.StopRide = function(callback)
{
sensors.Stop();
gpxWriter.EndFile();
clearInterval(sensorTimer);
States.SetCurrentState(States.States.RideStopped);
return "OK";
}
/**
* CancelRide
* simply stops sensors and sets state
*/
exports.CancelRide = function()
{
sensors.Stop();
States.SetCurrentState(States.States.Ready);
return "OK";
}
// updates the gpx file with new position data
var sensorReader = function(data)
{
checkSensors(data);
Data.recordCnt = ((Data.recordCnt + 1) % 2);
if ((PosData.bike.speed > 0) && (Data.recordCnt == 1))
{
gpxWriter.AddTrackPoint(PosData);
}
// if we "overshoot" end the gpx file and ride
if (Data.lastSegIdx > Data.segments.length)
{
StopRide();
}
}
// updates the resistance during ride
// everytime the resistance is changed we also will get sensor data... see: sensors.js
var rideinterval = function()
{
sensors.SetServoPos(PosData.power.resistance);
}
// --- Postion Ride and Sensor Calculations ---------------
var calcNewPoint = function(dist, lat1, lng1, lat2, lng2)
{
var pos1 = latlon(lat1, lng1);
var pos2 = latlon(lat2, lng2);
var bearing = pos1.bearingTo(pos2);
var newPos = pos1.destinationPoint(dist, bearing);
return { lat: newPos.lat, lng: newPos.lon }
}
var RecalcPos = function()
{
var seg = getSegment();
if (seg.distDriven > seg.dist)
{
PosData.pos.lat = seg.end.lat
PosData.pos.lng = seg.end.lng;
}
{
var newPos = calcNewPoint(seg.distDriven, seg.start.lat, seg.start.lng, seg.end.lat, seg.end.lng)
PosData.pos.lat = newPos.lat;
PosData.pos.lng = newPos.lng;
}
if (PosUpdateCallback)
{
PosUpdateCallback(PosData);
}
}
// uses sensor data to calculate new position and resistance
var checkSensors = function(sensorData)
{
PosData.bike.speed = calc.floor(sensorData.mps * 3.6, 2); // convert mps to kph
PosData.bike.pulse = sensorData.hr;
PosData.bike.cad = Math.floor(sensorData.cad);
var DistDriven = sensorData.dist;
var segment = getSegment(); // gets the current track segment we are in (inbetween two trackpoints)
if (segment)
{
// update current time/data (for gpx file)
PosData.time = new Date();
PosData.pos.ele = segment.ele;
PosData.pos.lat = segment.lat;
PosData.pos.lng = segment.lng;
PosData.pos.slope = segment.slope;
// calculates watts and resistance from speed slope and weight
PowerCalc.CalcPowerData(sensorData.mps, PosData.pos.slope);
// these are the watts we are able to generate with the trainer / the Watts we need to perform
PosData.power.watt = PowerCalc.PowerData.RealWatts+"/" +PowerCalc.PowerData.VirtualWatts;
// We only slowly in/decrease resistance (like in reality)
var res = PowerCalc.PowerData.Resistance - PosData.power.resistance;
if (Math.abs(res) > 0.1)
{
res = 0.1 *(Math.abs(res)/res);
}
// if the "virtual"/needed watts are more then we are currently able to produce via the trainer we cut it from the distance
if (PowerCalc.PowerData.RealWatts < PowerCalc.PowerData.VirtualWatts)
{
DistDriven = (DistDriven / PowerCalc.PowerData.VirtualWatts) * PowerCalc.PowerData.RealWatts;
}
segment.distDriven += DistDriven // we add the driven distance to the current segment data
// setting the "virtual data"
PosData.power.resistance +=res;
PosData.power.resPerc = calc.floor(PosData.power.resistance * 100, 0);
PosData.bike.virtualSpeed = calc.ceil(DistDriven * 3.6, 2);
// storing currently overall performance
PosData.LastDist = DistDriven;
Data.odo += DistDriven;
PosData.total = calc.floor(Data.segments.Odo/1000, 2);
PosData.odo = calc.floor(Data.odo/1000, 3);
// calculating current elevation from startpoint slope and driven distance
// (as we are between two trackpoints)
PosData.pos.ele = calc.floor(PosData.pos.ele + (PosData.pos.slope * (segment.end.ODO - Data.odo)/-10),1);
// same here for new trackpoints
RecalcPos(segment);
}
};
// will search for the current segment (between two track points) we are in
// needed to calculate resistance
var getSegment = function()
{
if (Data.lastSegIdx < Data.segments.length) // check if we drove over the last segment
{
var tmpIdx = Data.lastSegIdx;
var seg = Data.segments[tmpIdx];
// search for the new segment by checking if we allready drove the distance needed to successfully finish this segment
while (tmpIdx < Data.segments.length && seg.distDriven >= seg.dist)
{
tmpIdx++;
if (tmpIdx < Data.segments.length)
{
// if we finished the semgent we use the "leftover" distance to use for the new segment
var diff = seg.distDriven - seg.dist;
seg = Data.segments[tmpIdx];
seg.distDriven = diff;
}
}
// simple check if the new found segment is the last
if (tmpIdx >= 0)
{
Data.lastSegIdx = tmpIdx;
if (Data.lastSegIdx > Data.segments.length)
{
Data.lastSegIdx = Data.segments.length -1;
}
}
}
return Data.segments[Data.lastSegIdx];
}
// --- Mountain SVG Data ------
exports.GetMountain = function()
{
return SvgMountain();
}
// we create a profile of the elevation we are about to drive
var SvgMountain = function()
{
var path = "";
var size = { width: 1200, height: 150 }
var xratio = Math.ceil(Data.segments.Odo /size.width);
var height = Math.ceil(Data.segments.MaxEle-Data.segments.MinEle);
var yratio = Math.ceil(height / size.height);
var lastx = 0;
var yZero = height / yratio;
for (var i = 0; i < Data.segments.length; i++)
{
var seg = Data.segments[i];
var newx = Math.floor(seg.end.ODO / xratio);
if (newx > lastx)
{
lastx = newx;
path += " L "+newx+" "+calc.ceil((Data.segments.MaxEle - seg.ele) /yratio, 2);
}
}
var tmp = '<svg id="MountainSVG" internalwidth='+size.width+' width="'+size.width+'" viewBox="0 0 '+size.width+' '+size.height+'" xmlns="http://www.w3.org/2000/svg" version="1.1">';
tmp += '<path d="M 0 '+yZero;
tmp += path+'L '+size.width+' '+yZero+'" fill="gray" />';
tmp += '<rect id="svgBikePos" x="0" y="0" width="0.1" height="'+yZero+'" stroke="red" stroke-width="0.5"/>'
tmp +='</svg>';
return tmp;
}
<file_sep>/test/hardware/test_serial.py
#Fallback test for serial communication
import serial #test as serial
import time
import sys
def writeSerial(data):
port.write(data)
port.write("\n")
rcv = port.readline()
#bytesToRead = port.inWaiting()
#rcv = port.read(bytesToRead)
print rcv
comport = "/dev/ttyACM0"
port = serial.Serial(comport, baudrate=9600, timeout=3.0)
port.close()
port.open()
sin = raw_input("Pos: ")
while not (sin == "exit"):
print(sin)
writeSerial(sin)
sin = raw_input("Pos: ")
port.close()
<file_sep>/power/WattCalculator.js
/*
* Cycling power vs. velocity, based on a physical model.
*
* Copyright 2012 by <NAME> (gribble [at] gmail (dot) com)
* http://www.gribble.org/
*
* Many thanks again for allowing me to use your code!
*/
var Settings = require('../settings.js');
var WattCalculator = function()
{
this.Params = {
WeightRider : 75, // weight of rider (kg)
WeightEquipment : 8, // weight of bike (kg)
FrontArea : 0.55, // frontal area, rider+bike (m^2) (original 0,509)
DragCoeff : 0.7, // drag coefficient Cd (original 0,63)
DriveDrainLoss : 4, // drivetrain loss Loss_dt (original 3%)
RollingResistance : 0.005, // coefficient of rolling resistance Crr
AirDensity : 1.226, // air density (kg / m^3)
Slope : 0, // grade of hill (%)
Speed : 0 // speed in km/h
};
}
/**
* CalcPower
* uses the speed, slope and formula from Steve Gribble to calculate the power output needed at the wheels
*/
WattCalculator.prototype.CalcPower = function(speed, slope)
{
this.Params.WeightRider = Settings.Data.PowerCalc.RiderWeightKG;
this.Params.WeightEquipment = Settings.Data.PowerCalc.BikeWeightKG;
this.Params.FrontArea = Settings.Data.PowerCalc.FrontArea;
this.Params.DragCoeff = Settings.Data.PowerCalc.DragCoeff;
this.Params.DriveDrainLoss = Settings.Data.PowerCalc.DriveDrainLoss;
this.Params.RollingResistance = Settings.Data.PowerCalc.RollingResistance;
this.Params.Slope = slope;
this.Params.Speed = speed;
return CalculatePower(this.Params).Wheel;
}
// Calculates and returns the force components needed to achieve the
// given velocity. <params> is a dictionary containing the rider and
// environmental parameters, as returned by ScrapeParameters(), i.e.,
// all in metric units. 'velocity' is in km/h.
function CalculateForces(params)
{
// calculate Fgravity
var Fgravity = 9.8067 *
(params.WeightRider + params.WeightEquipment) *
Math.sin(Math.atan(params.Slope / 100.0));
// calculate Frolling
var Frolling = 9.8067 *
(params.WeightRider + params.WeightEquipment) *
Math.cos(Math.atan(params.Slope / 100.0)) *
(params.RollingResistance);
// calculate Fdrag
var Fdrag = 0.5 *
(params.FrontArea) *
(params.DragCoeff) *
(params.AirDensity) *
//(params.Speed) *// * 1000.0 / 3600.0) // check if speed squared is needed (air drag)!!!
(params.Speed);// * 1000.0 / 3600.0);
// cons up and return the force components
var ret = { };
ret.Fgravity = Fgravity;
ret.Frolling = Frolling;
ret.Fdrag = Fdrag;
return ret;
}
// Calculates and returns the power needed to achieve the given
// velocity. <params> is a dictionary containing the rider and
// environmenetal parameters, as returned by ScrapeParameters(), i.e.,
// all in metric units. 'velocity' is in km/h. Returns power in
// watts.
function CalculatePower(params)
{
// calculate the forces on the rider.
var forces = CalculateForces(params);
var totalforce = forces.Fgravity + forces.Frolling + forces.Fdrag;
// calculate necessary wheelpower
var wheelpower = totalforce * params.Speed;// * 1000.0 / 3600.0);
// calculate necessary legpower
var legpower = wheelpower / (1.0 - (params.DriveDrainLoss/100.0));
return { Leg: legpower, Wheel: wheelpower};
}
module.exports = WattCalculator;<file_sep>/power/PowerCalcPostCycla.js
/**
* Power Calculator for PostCycla equipment
* uses speed, slope, weight,.... to calculate the power/resistance needed to perform
*
* Calculates:
* Watts from speed, slope and weight in kg
*
* Resistance level from Watts and speed
* resistance shall be in percentage from max resistance of the equipment
* Values: 0.0 - 1.0
*
* Raw_Resistance
* resistance in percentage from maxResistance of equipment
* (was implemented because maximum resistance may be exceeded)
*
* Every fitness equipment needs to implement it's own Resistance level calculations
*
* Will create a power data object:
* RealWatts => the watts we are able to produce with the trainer (may be to small)
* VirtualWatts => the watts needed to perform (may exceed the resistance the trainer can provide)
* Resistance => the resistance for the trainer in percent (0..100%) this will be sent to the trainer
* Raw_Resistance => the resistance needed to perform in percent (0..xxx% may be more then we can provide)
*
* @author <NAME>
* @version 1.0
*/
var Settings = require('../settings.js');
var calc = require('../util/calc.js');
var WattsCalc = require('./WattCalculator.js');
// 160 Watts are needed when driving a -1% hill with 10 m/s
// calculated via https://www.gribble.org/cycling/power_v_speed.html
var MinimumJoulperMeter = 16;
/**
* PowerCalcPostCycla
* Uses the WattCalculator and a minimum setting to calculate power/resistance needed
*/
var PowerCalcPostCycla = function()
{
this.Watts = new WattsCalc();
this.PowerData =
{
RealWatts: 0,
VirtualWatts : 0,
Resistance : 0,
Raw_Resistance :0
};
}
/**
* PowerCalcPostCycla
* Uses the WattCalculator and a minimum setting to calculate power/resistance needed
*/
PowerCalcPostCycla.prototype.CalcPowerData = function(ms, slope)
{
this.CalcWatts(ms, slope);
this.CalcResistenceLevel(this.PowerData.VirtualWatts,ms);
return this.PowerData;
};
PowerCalcPostCycla.prototype.CalcResistenceLevel = function(watt, ms)
{
kmh = ms * 3.6
// This formula derived from the resistance measuring for a TACX CYCLETRAINER T1810
// see: http://www.fahrrad.de/fahrradzubehoer/rollentrainer-tacx-elite/tacx-cycletrainer-speedmatic-t1810/7146.html
// THIS NEEDS TO BE CALCULATED FOR EVERY TRAINER
var level = (((watt)-(0.05569*kmh+1.223)*kmh)/(1.3811*kmh-5.0809));
// multiply by 0,14285714285714285714285714285714
// since first calculations where done by tacx resistance (0-7) and we now want 0..100%
this.PowerData.Raw_Resistance = calc.ceil(level* Settings.Data.PowerCalc.PowerOffset* 0.14285714285714285714285714285714, 2) ;
// cutoff "overshot"
if (level < 1)
{
level = 1;
} else if (level > 7)
{
level = 7;
}
this.PowerData.Resistance = this.PowerData.Raw_Resistance;
// since the formula used can produce values of more/less then 0% - 100% we "cut off" wrong values.
if (this.PowerData.Resistance > 1)
{
this.PowerData.Resistance = 1;
this.PowerData.RealWatts = calc.floor((1.3811*kmh-5.0809)*level + (0.05569*kmh+1.2233)*kmh, 0);
} else if (this.PowerData.Resistance < 0)
{
this.PowerData.Resistance = 0;
this.PowerData.RealWatts = calc.floor((1.3811*kmh-5.0809)*level + (0.05569*kmh+1.2233)*kmh, 0);
}
};
// Convert Speed and slope to Power :
// Calculations used from: https://www.gribble.org/cycling/power_v_speed.html
PowerCalcPostCycla.prototype.CalcWatts = function(ms, slope)
{
this.PowerData.VirtualWatts = calc.ceil(this.Watts.CalcPower(ms, slope),0);
this.PowerData.RealWatts = this.PowerData.VirtualWatts;
};
// export the class
module.exports = PowerCalcPostCycla;
<file_sep>/HW_Controller/PostCyclaHardwareMock/PostCyclaHardwareMock.ino
/**
* Hardware Mockup for the PostCycla stm32 sensor and server controller
* Input:
* any character and newline as delimiter
* (will only evaluate inputs a-z so we are able to use the keyboard for debugging)
*
* Output:
* (will be triggered every time an input was sent)
* Will send a JSON string containing:
* { "distance" : xxx, "speedms" : xxx, "servoPos" : xxx, "charSent" : xxx }
*
* distance => dinstance since the last time the sensor was read
* speedms => current speed in meters per second
* servoPos => current position of the servo setting the trainer resistence
* sentChar => the servo position can be set from 0...100% and will be sent to the STM as an character followed by a newline
* this sentChar was implemented for debugging and will show the last sent character (should be between 32 and 132)
*
* for Debugging will use the a-z input to convert them into 0..10 and use this as an output for distance, speed and servoPos
*
* @author <NAME>
* @version 1.0
*/
char lastReceivedChar;
// main setup will just initialize the serial port
void setup()
{
Serial.begin(9600);
}
// main loop will read all data from serial and send back an answer
void loop()
{
recvOneChar();
}
// checks the serial input for
void recvOneChar()
{
if (Serial.available() > 0) // checks if anything can be read
{
char tmp = Serial.read();
if (tmp == '\n') // checks for newline delimiter
{
// convert input to value between 0..10
float serverPos = (float(lastReceivedChar) - 97)* 0.4;
if (serverPos > 10)
{
serverPos = 10;
} else if (serverPos < 0)
{
serverPos = 0;
}
//rturn output
Serial.print("{ \"distance\" : ");
Serial.print(serverPos);
Serial.print(", \"speedms\" : ");
Serial.print(serverPos);
Serial.print(", \"servoPos\" : ");
Serial.print(serverPos);
Serial.print(", \"sentChar\" : ");
Serial.print(int(lastReceivedChar));
Serial.println(" }");
} else
{
lastReceivedChar = tmp; // if not newline
}
}
}
<file_sep>/README.md
# PostCycla
Is a DIY project to create a smart bike trainer with "virtal ride" capability, using a Raspberry Pi and Node.js for the server and a Web UI on the client side. A STM32 MCU Nucleo with a CNY 70 sensor and a servo will get data from the trainer and set the resistance.
For testing and development mockup implementations of all sensors and actuators are available at: [Running the tests](#running-the-tests)
To set up sensors and actuators you can use any microcontroller to read/control sensors and a servo.
Every component was encapsulated to allow easy swapping of sensors, controllers or provide another way of sensing distance/speed and setting resistance on the trainer.
Future modules/components will include a way to directly read data and set resistance of Ant+ FE-C capable trainers.
## Getting Started
### On Windows
* Clone the repository
* Install the [Node modules](#installing)
* [Start everything](#how-to-start)
### On Raspberry
* Set up a Raspberry Pi with NodeJs.
* Clone the repository and copy it to the Raspberry
* Install the [Node modules](#installing)
* [Start everything](#how-to-start)
## Prerequisites
### Hardware
* Raspberry Pi 3
* STM32 MCU Nucleo/Arduino Nano
* CNY 70
* Servo
* Ant+ USB Stick
* Ant+ heart rate monitor chest strap
* Ant+ candence sensor
(the current implementation uses this hardware setup)
### Software
* NodeJs 4.8.2 (on Raspberry Pi)
Node Modules:
* [usb](https://github.com/tessel/node-usb)
* [serialport](https://www.npmjs.com/package/serialport#module_serialport--SerialPort+event_error)
* [xml2js](https://github.com/isaacs/sax-js/)
* [socket.io](https://socket.io/get-started/chat/)
* [express](http://expressjs.com/de/)
A gpx file for testing/running the software. **The gpx file needs to contain elevation data!**
### Installing
**USB**
```
sudo apt-get install build-essential libudev-dev
npm install usb
```
**Serial port**
```
sudo npm install serialport --unsafe-perm --build-from-source
```
**XML Parser**
```
npm install xml2js
```
**Web sockets**
```
npm install --save socket.io
```
**Express**
```
npm install express --save
```
## Running the tests
To test PostCycla itself you can set the "UseMockups" and "DebugOutput" flags in the "settings.json" file and [start the program](#running-the-program).
```
...
"UseMockups": true,
"DebugOutput": false,
...
```
In the "test" folder you will find test for the calculation and hardware components.
For hardware testing you can also use the [hardware mockup code](#arduino-hw-mockup).
**Calculations**
```
node test/calculations/PowerCalc_test.js
```
Tests the power calculation with a fixed speed and changing slope.
* Should provide a console output of the used speed, slope and calculated power data
```
node test/calculations/TrackParsingAndSmoothing_test.js
```
Tests the gpx file parser and smoothing algorithm
* Needs the original example/testing track gpx file (track segment length is fixed for testing)
* Should result in a console output either showing an error or the new smoothed segments
**Hardware**
```
node test/hardware/testConnections.js
```
Tests the Serial, USB and Ant+ hardware and connection to it
* Will try to find USB devices and the Ant+ Stick
* Will also try to connect to the SerialPort (ttyACM0 or COM3)
* Should return a console ouput regarding Testname, Success, and data/info about success or failure
```
node test/hardware/testAntplusSensors.js
```
This test will try and get data from an Ant+ stick with connected heart rate and cadence sensors
* Should return a console ouput with the heart rate and cadence (if sensors are connected to the Ant+ stick)
```
node test/hardware/testSTMSensor.js
```
This test will try and get data from the STM32/Arduino and check the output
* Should return a console ouput with the data from the module
For hardware testing you can also use the [hardware mockup code](#arduino-hw-mockup).
## Running the program
### Setup
To set up the hardware use the code in the "HW_Controller" folder.
#### STM32 MCU Nucleo
Contains the code currently used in the project
```
HW_Controller/mbed/main.cpp
```
#### Arduino HW mockup
Contains code for testing the hardware communication modules
```
HW_Controller/PostCyclaHardwareMock/PostCyclaHardwareMock.ino
```
#### Without hardware
Change the "settings.json" file:
```
...
"UseMockups": true,
"DebugOutput": true,
...
```
If these settings are used, you can run PostCycla without any hardware attached and get additional information in the Node.js console.
### How to start
```
sudo node postcycla.js
```
The web UI can be accessed via the address shown in the nodejs output.
Deault:
```
http://localhost:8125/
```
Simply open this address in your web browser to see the client UI.
* For testing an "Example_Testing Track.gpx" was added in the "tracks" folder.
* The new GPX will be generated in the "tracks" folder (will not be available in the UI)
**Note to finish/close the new GPX file you need to "STOP" the ride (even when you reached the end)**
## Built With
* [Node.JS](https://nodejs.org/) -
* [Visual Studio Code](https://code.visualstudio.com/) - IDE
## Contributing
Everyone is welcome to help, add, improve, ... only one rule here: "Don't be a dick".
## Authors
* **<NAME>** - *Initial idea / hardware / code* - [HackaDay project](https://hackaday.io/project/18658-postcycla)
## License
This project is licensed under the MIT License - see the [LICENSE.md](LICENSE.md) file for details.
## Acknowledgments
A big thanks to the following:
* [<NAME>](https://www.gribble.org/cycling/power_v_speed.html) - providing the power calculations
* [<NAME>](mourner.github.io/simplify-js) - and his Simplify.js scripts
* [Movable Type Ltd](http://www.movable-type.co.uk) - for their gps calculation scripts
* [<NAME> and Contributors](https://github.com/isaacs/sax-js/) - xml parsing for the gpx files
* [OpenLayers Map Viewer Library](https://openlayers.org/) - providing a solid map library
* [<NAME>](https://github.com/Loghorn/ant-plus) - Ant+ node module
* [Nonolith Labs, LLC](https://github.com/tessel/node-usb) - USB library for Node.JS
* [<NAME>](https://github.com/node-serialport/node-serialport) - Node Serialport
* [Socket.io](https://socket.io) - Web sockets
* [Express](http://expressjs.com/de/) - Web server<file_sep>/stm/newstm.js
/**
* STM32 sensor module
* This module connects via serial port to a connected STM32 board to read sensor data and set the servo position
* The STM32 should return the following data:
* { "distance" : 0, "speedms" : 0, "servoPos" : 0, "sentChar" : 0 }\n
*
* distance => dinstance since the last time the sensor was read
* speedms => current speed in meters per second
* servoPos => current position of the servo setting the trainer resistence
* sentChar => the servo position can be set from 0...100% and will be sent to the STM as an character followed by a newline
* this sentChar was implemented for debugging and will show the last sent character (should be between 32 and 132)
*
* Every sent or received data should be delimited with a newline
*
* The current hardware consists of a STM32 board with connected CNY70 reflex sensor to sense rotational speed of the trainers roller and a servo to move the bowden cable to set the resistance.
*
* @author <NAME>
* @version 1.0
*/
var Settings = require('../settings.js');
var SerialPort = require('serialport');
var events = require('events');
var calc = require('../util/calc.js');
var SerialPort = require('serialport');
var port = null;
const Readline = SerialPort.parsers.Readline; // newline delimiter parser for the serialport
const parser = new Readline();
var ServoOffset = { Min : 0.2, Max: 0.9}; // max travel of the servo,... needs to be checked and set for every hardware
/**
* Constuctor
* Creates a new STM instance connecting to the set serial port
*/
var STM = function(comPort)
{
events.EventEmitter.call(this);
var me = this;
port = new SerialPort(comPort, { baudRate: 9600, autoOpen: false });
this.SensorReady = function()
{
this.emit('SensorReady');
}
// gets every data received will parse it and trigger a "SensorData" event to forward the parsed data
this.SensorData = function(strdata)
{
try
{
var data = JSON.parse(strdata);
// fallback since sometimes distance was emtpy !?? (needs to be checked on STM side)
if (data && data.distance == 0 && data.speedms > 0)
{
data.distance = data.speedms;
}
this.emit('SensorData', data);
} catch(e)
{
}
}
// Switches the port into "flowing mode"
parser.on('data', function(data)
{
if (Settings.Data.DebugOutput)
{
console.log('Data: ' + data);
}
if (data.trim() != "")
{
me.SensorData(data);
}
});
}
STM.prototype.__proto__ = events.EventEmitter.prototype;
/**
* Init
* Initializes the module and opens the serial port
*/
STM.prototype.Init = function()
{
ServoOffset = typeof Settings.Data.Sensors.ServoOffset !== 'undefined' ? Settings.Data.Sensors.ServoOffset : ServoOffset;
ServoOffset.Delta = ServoOffset.Max - ServoOffset.Min;
var me = this;
port.pipe(parser);
port.open(function (err)
{
if (err)
{
return console.log('Error opening COM port: ', err.message);
}
me.SensorReady();
});
}
/**
* Connect
* Initializes the module and opens the serial port
*/
STM.prototype.Connect = function()
{
this.Init();
}
/**
* Disconnect
* closes the serial port
*/
STM.prototype.Disconnect = function()
{
port.close();
}
/**
* SetLevel
* Sets the new resistance level (between 0..1) and sends it to the STM32
* should trigger a response from the SMT32
*/
STM.prototype.SetLevel = function(pos)
{
// servo controller expects values from 32 to 132 (0..100%)
pos = checkServoEndpointspos(pos);
var position = pos;
pos = (pos *100) +32;
// every message has to be delimmited by an newline
var send = String.fromCharCode(pos)+ '\n';
if (Settings.Data.DebugOutput)
{
console.log("Char: %s Pos: %d CHR: %d", send, position, send.charCodeAt(0));
}
port.write(send, 'ascii', function()
{
if (Settings.Data.DebugOutput)
{
console.log('data written');
}
});
}
// determined by enpoints of trainer bowden cable
var checkServoEndpointspos = function(pos)
{
var offestPos = calc.ceil(pos /1 * ServoOffset.Delta);
offestPos += ServoOffset.Min;
if (offestPos > ServoOffset.Max)
{
offestPos = ServoOffset.Max;
}
if (offestPos < ServoOffset.Min)
{
offestPos = ServoOffset.Min;
}
return offestPos;
}
// export the class
module.exports = STM; | 494bb009cec9de575068700f56fcd7736e0db048 | [
"JavaScript",
"Python",
"C++",
"Markdown"
] | 21 | JavaScript | jasonwiener/PostCycla | 3e13894de7f07f0d21aff249d686ceccc9ebbcd2 | 0bb878776b3ff68a86f100a668725c2372d5112a | |
refs/heads/master | <repo_name>melo1024/flaskWeb<file_sep>/app.py
# -*- coding:utf-8 -*-
""" 本地测试url
初始化:
http://127.0.0.1:5000/es/init?min_score=94&max_score=94&split_score=76
统计信息:
http://127.0.0.1:5000/all/count
球员数量统计:
http://127.0.0.1:5000/player/count
国家列表:
http://127.0.0.1:5000/nation/list
联赛列表:
http://127.0.0.1:5000/league/list
俱乐部列表:
http://127.0.0.1:5000/club/list?leagueName=美国足球职业大联盟
天赋列表:
http://127.0.0.1:5000/skill/list
活动列表:
http://127.0.0.1:5000/program/list
天赋等级提升(扑救大师):
http://127.0.0.1:5000/skill/updata?code=5581e0a0-00d1-11e8-9a00-8105ee8c8a87
位置等级提升:
http://127.0.0.1:5000/job/updata?code=ST
青云对象存储:
http://127.0.0.1:5000/file
网站首页:
http://127.0.0.1:5000/fifamobile
"""
from flask import Flask, Response, send_file, send_from_directory, make_response
from flask import request, render_template
import esHandler
import qingstorHandler
from io import BytesIO
import tempfile
import os
"""Flask app"""
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello World!'
@app.route('/fifamobile')
def fifa_mobile():
return render_template("players.html")
@app.route('/file')
def return_file():
key = request.args.get('key')
r = qingstorHandler.getRespByKey(key)
b = bytes()
for chunk in r.iter_content():
b = b + chunk
response = make_response(b)
response.headers["Content-Disposition"] = "attachment; filename={}".format(key)
return response
@app.route('/es/init', methods=['get'])
def init_es():
min_score = request.args.get('min_score')
max_score = request.args.get('max_score')
split_score = request.args.get('split_score')
if min_score is None or max_score is None or split_score is None:
return 'Invalid request, pls check the url.'
if min_score.isdigit() and max_score.isdigit() and split_score.isdigit():
# # 初始化国家
# esHandler.initNationIndex()
# # 初始化联赛/俱乐部
# esHandler.initLeagueAndClubIndex()
# # 初始化天赋
# esHandler.initSkillIndex()
# # 初始化活动
# esHandler.initProgramIndex()
# # 初始化位置等级提升
# esHandler.initJobLevelCapabilityIndex()
# 初始化球员
esHandler.initPlayerIndex(int(min_score), int(max_score), int(split_score))
return 'ES init complete!'
else:
return 'Param type error.'
@app.route('/all/count', methods=['get'])
def countAll():
return esHandler.countAll()
@app.route('/player/count', methods=['get'])
def count():
return esHandler.countPlayers()
@app.route('/player/list', methods=['get'])
def searchPlayer():
print(request.args)
min_score = request.args.get('min_score')
max_score = request.args.get('max_score')
name = request.args.get('name')
nation = request.args.get('nation')
league = request.args.get('league')
club = request.args.get('club')
job = request.args.get('job')
skill = request.args.get('skill')
#活动,比如黄金周/七夕
program = request.args.get('program')
result = esHandler.searchPlayers(min_score, max_score, name, nation, league, club, job, skill, program)
return result
@app.route('/nation/list', methods=['get'])
def searchNation():
result = esHandler.searchNation()
return result
@app.route('/league/list', methods=['get'])
def searchLeague():
result = esHandler.searchLeague()
return result
@app.route('/club/list', methods=['get'])
def searchClub():
leagueName = request.args.get('leagueName')
result = esHandler.searchClub(leagueName)
return result
@app.route('/skill/list', methods=['get'])
def searchSkill():
result = esHandler.searchSkill()
return result
@app.route('/program/list', methods=['get'])
def searchProgram():
result = esHandler.searchProgram()
return result
@app.route('/job/updata', methods=['get'])
def searchJobLevelCapability():
job = request.args.get('code')
result = esHandler.searchJobLevelCapability(job)
return result
@app.route('/skill/updata', methods=['get'])
def searchSkillLevelCapability():
skill = request.args.get('code')
result = esHandler.searchSkillLevelCapability(skill)
return result
if __name__ == '__main__':
app.run()
<file_sep>/simpleApp.py
# -*- coding:utf-8 -*-
from flask import Flask, render_template
"""Flask app"""
app = Flask(__name__)
@app.route('/fifamobile')
def fifa_mobile():
return render_template("players.html")
@app.route('/test')
def test():
return 'ok'
if __name__ == '__main__':
app.run(host='0.0.0.0', port=80)<file_sep>/test.py
import requests
r = requests.get('http://img.wantuole.com/resources/backgrouds/VIP2.png')
print(r.content)<file_sep>/static/js/common.js
$(function() {
initParts();
setTimeout(function() {
initCommonModule();
}, 1000);
})
function initParts() {
$("#banner").load("parts/banner.html");
$("#functions").load("parts/functions.html");
}
function initCommonModule() {
ajax.get('count?code=fifamobile', function(data) {
$("#cardTotal").text(data.cardTotal);
});
}
/**
* 调用html2canvas框架进行截图下载功能
* @param domId 容器Id
* author Levin
*/
function domShot(domId) {
//0.5.0-beta4方法
html2canvas(document.querySelector("#" + domId), {
allowTaint: true,
height: $("#" + domId).outerHeight() + 20
}).then(function(canvas) {
var timestamp = Date.parse(new Date());
$('#down_button').attr('href', canvas.toDataURL());
$('#down_button').attr('download', timestamp + '.png');
var fileObj = document.getElementById("down_button");
fileObj.click();
});
}
function getBackground(pn, r, score, hb, bg) {
var imgUrl = "http://img.wantuole.com/resources/backgrouds/";
var num = 0;
var imgName = "";
if(parseInt(hb) > 0) {
num = parseInt((parseInt(score) - 70) / 10);
if(bg == "CGER" || bg == 'SC' || bg == 'TC' || bg == 'T' || bg == 'TDYX' || pn.indexOf('剧情精英球员') >= 0 || bg == 'ZCBase')
num = parseInt((parseInt(score) - 50) / 10);
else if(bg == 'JLBZ')
num = parseInt((parseInt(score) - 60) / 10);
if(pn == '世界杯球员') {
if(num > 4) {
num = 4;
}
}
imgName = bg + num + ".png";
} else {
num = parseInt((parseInt(score) - 50) / 10);
if(bg != "BASIC") {
imgName = bg + num + ".png";
} else {
switch(parseInt(r)) {
case 1:
imgName = "";
break;
case 2:
imgName = "MR";
break;
case 3:
imgName = "ER";
break;
case 4:
imgName = "GR";
break;
case 5:
imgName = "SR";
break;
case 6:
imgName = "BR";
break;
}
imgName = imgName + num + ".png";
}
}
imgUrl = imgUrl + imgName;
return imgUrl;
}
function getHead(pn, no, score, calcscore, head, count, haveas) {
var imgUrl = "http://img.wantuole.com/FIFA%E8%B6%B3%E7%90%83%E4%B8%96%E7%95%8C/players/";
if(pn == '世界杯球员') {
var imgUrl = "http://img.wantuole.com/FIFA%E8%B6%B3%E7%90%83%E4%B8%96%E7%95%8C/wcplayers/";
}
var num = 0;
var imgName = "";
if(parseInt(haveas) == 1) {
if(parseInt(score) >= 80) {
if(calcscore)
imgName = no + "_AS" + calcscore + ".png";
else
imgName = no + "_AS.png";
} else {
imgName = no + ".png";
}
} else {
if(head == "TOTW") {
if(score) {
imgName = no + "_" + head + calcscore + ".png";
} else {
imgName = no + "_" + head + ".png";
}
} else {
if(parseInt(count) > 1) {
num = parseInt((parseInt(score) - calcscore) / 10);
if(head == "IC" || head == "PI") {
if(num == 0)
num = 1;
}
if(num > count)
num = count;
imgName = no + "_" + head + num + ".png";
} else {
if(head) {
if(parseInt(score) >= 80) {
imgName = no + "_" + head + ".png";
if(head == 'MM') {
if(score >= 100) {
imgName = no + "_L" + head + ".png";
}
} else if(head == 'LVL') {
if(score >= 100) {
imgName = no + "_LVLM.png";
}
}
} else {
if(calcscore == -1) {
imgName = no + "_" + head + ".png";
} else {
imgName = no + ".png";
}
}
} else
imgName = no + ".png";
}
}
}
imgUrl = imgUrl + imgName;
return imgUrl;
}
function getBasicValues(job, values) {
var basicValues = [];
if(job != 'GK') {
basicValues[0] = Math.floor(values[1] * 0.45 + values[0] * 0.55);
basicValues[1] = Math.floor(values[6] * 0.45 + values[7] * 0.20 + values[8] * 0.25 + values[16] * 0.1);
basicValues[2] = Math.floor(values[17] * 0.45 + values[18] * 0.25 + values[19] * 0.3);
basicValues[3] = Math.floor(values[4] * 0.55 + values[3] * 0.3 + values[15] * 0.15);
basicValues[4] = Math.floor(values[11] * 0.50 + values[10] * 0.35 + values[5] * 0.15);
basicValues[5] = Math.floor(values[2] * 0.6 + values[12] * 0.4);
} else {
basicValues[0] = values[6];
basicValues[1] = values[7];
basicValues[2] = values[9];
basicValues[3] = values[8];
basicValues[4] = Math.round(values[17] * 0.35 + values[18] * 0.3 + values[19] * 0.3 + values[16] * 0.05);
basicValues[5] = Math.round(values[2] * 0.6 + values[12] * 0.4);
}
return basicValues;
}
//方法一:逐个字符检查是否中文字符
String.prototype.getByteLen = function() {
var len = 0;
for(var i = 0; i < this.length; i++) {
if((this.charCodeAt(i) & 0xff00) != 0)
len++;
len++;
}
return len;
}<file_sep>/static/js/playerContrast.page.js
var selectedRaritie;
var selectedNation;
var selectedJob;
var leagues;
var selectedLeague;
var selectedClub;
var selectedProgram;
var selectedProgramChild = '';
var selectedSkill;
var programs = [];
var cards = [];
var filter = false;
var filterCards;
var index = 1;
var size = 30;
var dataTable;
var selectedPlayer;
var players = [];
var playerHead = [];
var playerInfo = [];
var maxValue = [];
$(function() {
initPage();
})
function initPage() {
$("#raritySelect").multiselect({
includeSelectAllOption: false,
selectAllText: ' 全选',
nonSelectedText: '全部', //没有值的时候button显示值
nSelectedText: '个被选中', //有n个值的时候显示n个被选中
allSelectedText: '全选', //所有被选中的时候 全选(n)
enableFiltering: false,
numberDisplayed: 1,
filterPlaceholder: '查询',
onChange: function(option, checked, select) {
if(checked) {
selectedRaritie = $(option).val();
} else {
selectedRaritie = "";
}
}
});
$("#jobSelect").multiselect({
maxHeight: 500,
includeSelectAllOption: false,
selectAllText: ' 全选',
nonSelectedText: '全部', //没有值的时候button显示值
nSelectedText: '个被选中', //有n个值的时候显示n个被选中
allSelectedText: '全选', //所有被选中的时候 全选(n)
enableFiltering: false,
numberDisplayed: 1,
filterPlaceholder: '查询',
onChange: function(option, checked, select) {
if(checked) {
selectedJob = $(option).val();
} else {
selectedJob = "";
}
}
});
if(localStorage["nation"]) {
var data = JSON.parse(localStorage["nation"]);
var select = $('#nationSelect');
data.forEach(function(item, i) {
option = $("<option value='" + item.code + "' icon='" + item.icon + "' name='" + item.chname + "'>" + item.chname + "</option>");
option.appendTo(select);
});
select.multiselect({
maxHeight: 500,
includeSelectAllOption: false,
enableFiltering: true,
filterPlaceholder: '查询',
nonSelectedText: '请选择...',
onChange: function(option, checked, select) {
if(checked) {
selectedNation = $(option).val();
} else {
selectedNation = "";
}
}
});
} else {
ajax.get('nation/list', function(result) {
if(result.data && typeof(result.data) != undefined) {
localStorage.setItem("nation", JSON.stringify(result.data));
}
var select = $('#nationSelect');
result.data.forEach(function(item, i) {
option = $("<option value='" + item.code + "' icon='" + item.icon + "' name='" + item.chname + "'>" + item.chname + "</option>");
option.appendTo(select);
});
select.multiselect({
maxHeight: 500,
includeSelectAllOption: false,
enableFiltering: true,
filterPlaceholder: '查询',
nonSelectedText: '请选择...',
onChange: function(option, checked, select) {
if(checked) {
selectedNation = $(option).val();
} else {
selectedNation = "";
}
}
});
});
}
//localStorage.removeItem("league")
if(localStorage["league"]) {
var data = JSON.parse(localStorage["league"]);
var select = $('#leagueSelect');
data.forEach(function(item, i) {
var option = $("<option value='" + item.code + "'>" + item.chname + "</option>");
option.appendTo(select);
});
select.multiselect({
maxHeight: 500,
includeSelectAllOption: false,
enableFiltering: true,
filterPlaceholder: '查询',
onChange: function(option, checked, select) {
if(checked) {
selectedLeague = $(option).val();
} else {
selectedLeague = "";
}
selectedClub = "";
bindClub($(option).val());
}
});
bindClub('');
} else {
ajax.get('league/list', function(result) {
if(result.data && typeof(result.data) != undefined) {
localStorage.setItem("league", JSON.stringify(result.data));
}
var select = $('#leagueSelect');
result.data.forEach(function(item, i) {
var option = $("<option value='" + item.code + "'>" + item.chname + "</option>");
option.appendTo(select);
});
select.multiselect({
maxHeight: 500,
includeSelectAllOption: false,
enableFiltering: true,
filterPlaceholder: '查询',
onChange: function(option, checked, select) {
if(checked) {
selectedLeague = $(option).val();
} else {
selectedLeague = "";
}
selectedClub = "";
bindClub($(option).val());
}
});
bindClub('');
});
}
localStorage.removeItem("program")
if(localStorage["program"]) {
var data = JSON.parse(localStorage["program"]);
var select = $('#programSelect');
programs = data;
programs.forEach(function(item, i) {
if(item.isShow) {
if(item.isShow == "1") {
var option = $("<option value='" + item.chname + "'>" + item.chname + "</option>");
option.appendTo(select);
}
}
});
select.multiselect({
maxHeight: 500,
includeSelectAllOption: false,
enableFiltering: true,
filterPlaceholder: '查询',
nonSelectedText: '请选择...',
onChange: function(option, checked, select) {
if(checked) {
selectedProgram = $(option).val();
programs.forEach(function(program, index) {
if(program.chname == selectedProgram) {
if(program.childs) {
if(program.childs.length > 0) {
bindProgramChild(program.childs);
$("#programChildDiv").show();
} else {
selectedProgramChild = "";
$("#programChildDiv").hide();
}
} else {
selectedProgramChild = "";
$("#programChildDiv").hide();
}
}
});
} else {
selectedProgram = "";
}
}
});
} else {
ajax.get('program/list?gameCode=fifamobile', function(result) {
if(result.data && typeof(result.data) != undefined) {
localStorage.setItem("program", JSON.stringify(result.data));
}
var select = $('#programSelect');
programs = result.data;
programs.forEach(function(item, i) {
if(item.isShow) {
if(item.isShow == "1") {
var option = $("<option value='" + item.chname + "'>" + item.chname + "</option>");
option.appendTo(select);
}
}
});
select.multiselect({
maxHeight: 500,
includeSelectAllOption: false,
enableFiltering: true,
filterPlaceholder: '查询',
nonSelectedText: '请选择...',
onChange: function(option, checked, select) {
if(checked) {
selectedProgram = $(option).val();
programs.forEach(function(program, index) {
if(program.chname == selectedProgram) {
if(program.childs) {
if(program.childs.length > 0) {
bindProgramChild(program.childs);
$("#programChildDiv").show();
} else {
selectedProgramChild = "";
$("#programChildDiv").hide();
}
} else {
selectedProgramChild = "";
$("#programChildDiv").hide();
}
}
});
} else {
selectedProgram = "";
}
}
});
});
}
//localStorage.removeItem("skill")
if(localStorage["skill"]) {
var data = JSON.parse(localStorage["skill"]);
var select = $('#skillSelect');
data.forEach(function(item, i) {
var option = $("<option value='" + item.code + "'>" + item.name + "</option>");
option.appendTo(select);
});
select.multiselect({
maxHeight: 500,
includeSelectAllOption: false,
enableFiltering: true,
filterPlaceholder: '查询',
nonSelectedText: '请选择...',
onChange: function(option, checked, select) {
if(checked) {
selectedSkill = $(option).val();
} else {
selectedSkill = "";
}
}
});
} else {
ajax.get('skill/list?gameCode=fifamobile', function(result) {
if(result.data && typeof(result.data) != undefined) {
localStorage.setItem("skill", JSON.stringify(result.data));
}
var select = $('#skillSelect');
result.data.forEach(function(item, i) {
var option = $("<option value='" + item.code + "'>" + item.name + "</option>");
option.appendTo(select);
});
select.multiselect({
maxHeight: 500,
includeSelectAllOption: false,
enableFiltering: true,
filterPlaceholder: '查询',
nonSelectedText: '请选择...',
onChange: function(option, checked, select) {
if(checked) {
selectedSkill = $(option).val();
} else {
selectedSkill = "";
}
}
});
});
}
$("#sortSelect").multiselect({
maxHeight: 500,
includeSelectAllOption: false,
selectAllText: ' 全选',
nonSelectedText: '全部', //没有值的时候button显示值
nSelectedText: '个被选中', //有n个值的时候显示n个被选中
allSelectedText: '全选', //所有被选中的时候 全选(n)
enableFiltering: false,
numberDisplayed: 1,
filterPlaceholder: '查询',
onChange: function(option, checked, select) {
if(checked) {
if($(option).val()) {
dataTable.order([parseInt($(option).val()), "desc"]).draw();
}
}
}
});
//bindData();
$('#playerList tbody').on('click', 'tr', function() {
var data = dataTable.row(this).data();
buildInfo(data.code);
$('#playerSelect').modal('hide');
});
}
function bindClub(lcode) {
//localStorage.removeItem("club")
if(localStorage["club"] && lcode == '') {
var data = JSON.parse(localStorage["club"]);
var select = $('#clubSelect');
select.empty();
var option = $("<option value=''>全部</option>");
option.appendTo(select);
data.forEach(function(item, i) {
var option = $("<option value='" + item.code + "'>" + item.chname + "</option>");
option.appendTo(select);
});
select.multiselect('destroy').multiselect({
maxHeight: 500,
includeSelectAllOption: false,
enableFiltering: true,
filterPlaceholder: '查询',
nonSelectedText: '请选择...',
onChange: function(option, checked, select) {
if(checked) {
selectedClub = $(option).val();
} else {
selectedClub = "";
}
}
});
} else {
ajax.get('club/list?lcode=' + lcode, function(result) {
if(result.data && typeof(result.data) != undefined && lcode == '') {
localStorage.setItem("club", JSON.stringify(result.data));
}
var select = $('#clubSelect');
select.empty();
var option = $("<option value=''>全部</option>");
option.appendTo(select);
result.data.forEach(function(item, i) {
var option = $("<option value='" + item.code + "'>" + item.chname + "</option>");
option.appendTo(select);
});
select.multiselect('destroy').multiselect({
maxHeight: 500,
includeSelectAllOption: false,
enableFiltering: true,
filterPlaceholder: '查询',
nonSelectedText: '请选择...',
onChange: function(option, checked, select) {
if(checked) {
selectedClub = $(option).val();
} else {
selectedClub = "";
}
}
});
});
}
}
function bindProgramChild(childs) {
var select = $('#programChildSelect');
select.empty();
var option = $("<option value=''>全部</option>");
option.appendTo(select);
childs.forEach(function(item, i) {
var option = $("<option value='" + item.chname + "'>" + item.chname + "</option>");
option.appendTo(select);
});
select.multiselect('destroy').multiselect({
maxHeight: 500,
includeSelectAllOption: false,
enableFiltering: true,
filterPlaceholder: '查询',
nonSelectedText: '请选择...',
onChange: function(option, checked, select) {
if(checked) {
selectedProgramChild = $(option).val();
} else {
selectedProgramChild = "";
}
}
});
}
function bindData() {
$("#selectFilter").hide();
$("#wailt").show();
$("#playerList").hide();
var args = "";
var name = $("#name").val();
if(name) {
args += '&n=' + encodeURI(name);
}
if(selectedRaritie) {
args += '&r=' + selectedRaritie;
}
if(selectedJob) {
args += '&j=' + selectedJob;
}
if(selectedNation) {
args += '&nc=' + selectedNation;
}
if(selectedLeague) {
args += '&lc=' + selectedLeague;
}
if(selectedClub) {
args += '&cc=' + selectedClub;
}
if(selectedProgram) {
var program = selectedProgram;
if(selectedProgramChild) {
program = selectedProgram + "-" + selectedProgramChild;
}
args += '&pc=' + encodeURI(program);
}
if(selectedSkill) {
args += '&b=' + selectedSkill;
}
var foot = $("#footSelect").val();
if(foot) {
args += '&f=' + encodeURI(foot);
}
var height = $("#height").val();
if(height) {
args += '&h=' + h;
}
var scoreMin = $("#scoreMin").val();
var scoreMax = $("#scoreMax").val();
if(scoreMin || scoreMax) {
if(scoreMin) {
args += '&mi=' + scoreMin;
}
if(scoreMax) {
args += '&ma=' + scoreMax;
}
}
ajax.get('fifa/list2?1=1' + args, function(result) {
cards = result.data;
$("#wailt").hide();
$("#playerList").show();
buildCardList(cards)
});
}
function buildInfo(code) {
var max = 5;
if(!IsPC()) {
max = 3;
}
if(players.length < max) {
ajax.get('fifamobile/card/detail?code=' + code, function(data) {
ajax.get('levelup/list?gameCode=fifamobile&type=1&code=' + data.basicInfo.jobCode + '&level=' + data.basicInfo.score, function(result) {
data.train = {};
result.data.forEach(function(item) {
data.train[item.level] = {};
data.train[item.level].values = item.capabilityValues;
})
ajax.get('levelup/list?gameCode=fifamobile&type=2&code=' + data.skills.code + '&level=0', function(result) {
data.boost = {};
result.data.forEach(function(item) {
data.boost[item.level] = {};
data.boost[item.level].values = item.capabilityValues;
})
data.values = [];
for(var i = 0; i < 20; i++) {
data.values.push(data.capabilityValues.extend[i]);
}
data.selectTrain = data.basicInfo.score;
data.selectBoost = 0;
players.push(data);
compare();
});
});
});
}
}
function deletePlayer(i) {
players.splice(i, 1);
compare();
}
function compare() {
if(players.length == 0) {
$("#msg").show();
$("#compare").hide();
} else {
$("#msg").hide();
$("#compare").show();
}
$("div[type]").show();
for(var i = 0; i < 28; i++) {
maxValue[i] = 0;
}
var heads = $("#heads");
var infos = $("#infos");
playerHead.forEach(function(item, i) {
item.remove();
});
playerInfo.forEach(function(item, i) {
item.remove();
});
players.forEach(function(player, i) {
valueRefresh(player.code);
})
players.forEach(function(player, i) {
var headBox = $('<div class="col-sm-2 col-xs-3 compare-column"></div>');
var c = 'compare';
if(!IsPC()) {
c = 'mcompare'
}
var item = $("<div class='fm-card-img " + c + "'><img id='bg_" + player.code + "' class='background' width='100%' src='" +
getBackground(player.basicInfo.programInfo.name, player.rarityValue, parseInt(player.selectTrain) + parseInt(player.selectBoost), player.basicInfo.programInfo.havebackground, player.basicInfo.bg) +
"'><img id='head_" + player.code + "' class='player-img' width='100%' src='" +
getHead(player.basicInfo.programInfo.name, player.basicInfo.no, parseInt(player.selectTrain) + parseInt(player.selectBoost), player.basicInfo.headcalcscore, player.basicInfo.head, player.basicInfo.headcount, player.basicInfo.havaas) + "'></div>");
item.appendTo(headBox);
item = $('<h5>' + player.name + '</h5>');
item.appendTo(headBox);
item = $('<button type="button" class="btn red btn-xs margin-bottom-10" onclick="deletePlayer(' + i + ')">删除球员</button>');
item.appendTo(headBox);
var levelSelect = '<select id="train_' + player.code + '" class="form-control">';
for(var i = parseInt(player.basicInfo.score); i <= 100; i++) {
levelSelect += '<option value="' + i + '">' + i + '</option>';
}
levelSelect += '</select>';
item = $('<div class="compare-row compare-label">' + levelSelect + '</div>');
item.appendTo(headBox)
var skillSelect = '<select id="boost_' + player.code + '" class="form-control">';
for(var i = 0; i <= 20; i++) {
skillSelect += '<option value="' + i + '">' + i + '</option>';
}
skillSelect += '</select>';
item = $('<div class="compare-row compare-label">' + skillSelect + '</div>');
item.appendTo(headBox)
headBox.appendTo(heads);
playerHead.push(headBox);
var infoBox = $('<div class="col-sm-2 col-xs-3 compare-column"></div>');
item = $('<div class="compare-row compare-label"></div>');
item.appendTo(infoBox)
item = $('<div id="score_' + player.code + '" type="info" class="compare-row compare-label">' + (parseInt(player.selectTrain) + parseInt(player.selectBoost)) + '</div>');
item.appendTo(infoBox)
item = $('<div type="info" class="compare-row compare-label">' + player.basicInfo.job + '</div>');
item.appendTo(infoBox)
item = $('<div type="info" class="compare-row compare-label">' + (player.basicInfo.height ? player.basicInfo.height + 'cm' : '-') + '</div>');
item.appendTo(infoBox)
item = $('<div type="info" class="compare-row compare-label">' + (player.basicInfo.foot ? player.basicInfo.foot : '-') + '</div>');
item.appendTo(infoBox)
item = $('<div type="info" class="compare-row compare-label">' + player.skills.name + '</div>');
item.appendTo(infoBox)
item = $('<div type="info" class="compare-row compare-label">' + (player.basicInfo.traits ? player.basicInfo.traits : '') + '</div>');
item.appendTo(infoBox)
item = $('<div class="compare-row compare-label"><div></div></div>');
item.appendTo(infoBox)
var total = 0;
values = getBasicValues(player.basicInfo.jobCode, player.values)
for(var i = 0; i < 6; i++) {
total += parseInt(values[i]);
item = $('<div id="value' + i + '_' + player.code + '" type="basic" class="compare-row compare-label" index="' + i + '" data="' + values[i] + '">' + values[i] + '</div>');
if(maxValue[i] < parseInt(values[i])) {
maxValue[i] = parseInt(values[i]);
}
item.appendTo(infoBox);
}
item = $('<div id="value6_' + player.code + '" type="basic" class="compare-row compare-label" index="6" data="' + total + '">' + total + '</div>');
if(maxValue[6] < total) {
maxValue[6] = total;
}
item.appendTo(infoBox);
item = $('<div class="compare-row compare-label"></div>');
item.appendTo(infoBox);
total = 0;
for(var i = 0; i < 20; i++) {
total += parseInt(player.values[i]);
item = $('<div id="value' + (i + 7) + '_' + player.code + '" type="extend" class="compare-row compare-label" index="' + (i + 7) + '" data="' + player.values[i] + '">' + player.values[i] + '</div>');
if(maxValue[i + 7] < parseInt(player.values[i])) {
maxValue[i + 7] = parseInt(player.values[i]);
}
item.appendTo(infoBox)
}
item = $('<div id="value27_' + player.code + '" type="extend" class="compare-row compare-label" index="27" data="' + total + '">' + total + '</div>');
if(maxValue[27] < total) {
maxValue[27] = total;
}
item.appendTo(infoBox)
infoBox.appendTo(infos);
playerInfo.push(infoBox);
$("#train_" + player.code).val(player.selectTrain);
$("#train_" + player.code).bind('change', function() {
player.selectTrain = $("#train_" + player.code).val();
playerInfoChange(player.code);
});
$("#boost_" + player.code).val(player.selectBoost);
$("#boost_" + player.code).bind('change', function() {
player.selectBoost = $("#boost_" + player.code).val();
playerInfoChange(player.code);
});
});
$(".compare-label[index]").addClass("font-grey-salt");
for(var i = 0; i < 28; i++) {
$(".compare-label[index='" + i + "'][data='" + maxValue[i] + "']").addClass("font-red bold");
}
}
function playerInfoChange(code) {
compare();
}
function valueRefresh(code) {
players.forEach(function(player, i) {
if(player.code == code) {
player.values = [];
for(var i = 0; i < 20; i++) {
player.values.push(player.capabilityValues.extend[i]);
}
for(var i = parseInt(player.basicInfo.score) + 1; i <= parseInt(player.selectTrain); i++) {
for(var j = 0; j < 20; j++) {
if(parseInt(player.values[j]) + parseInt(player.train[i].values[j]) > 120) {
player.values[j] = 120;
} else {
player.values[j] = parseInt(player.values[j]) + parseInt(player.train[i].values[j]);
}
}
}
if(parseInt(player.selectBoost) > 0) {
for(var i = 0; i < 20; i++) {
if(parseInt(player.boost[parseInt(player.selectBoost)].values[i]) > 0) {
var value = parseInt(player.values[i]);
value = value + Math.round(parseInt(player.values[i]) * parseInt(player.boost[parseInt(player.selectBoost)].values[i]) / 100);
if(value > 140) {
player.values[i] = 140;
} else {
player.values[i] = value;
}
}
}
}
}
});
}
function buildCardList(cards) {
if(dataTable) {
dataTable.clear();
dataTable.destroy();
}
cards.forEach(function(card, i) {
var values = getBasicValues(card.basicInfo.jobCode, card.capabilityValues.extend);
card.values = values;
});
dataTable = $('#playerList').DataTable({
"data": cards,
"deferRender": true,
"destroy": true,
columnDefs: [{
targets: 0,
orderable: false,
width: "10%",
render: function(data, type, row, meta) {
return "<div class='fm-card-img'><img class='background' height='60' src='" +
getBackground(row.basicInfo.programInfo.name, row.rarityValue, row.basicInfo.score, row.basicInfo.programInfo.havebackground, row.basicInfo.bg) +
"'><img class='player-img' height='60' src='" +
getHead(row.basicInfo.programInfo.name, row.basicInfo.no, row.basicInfo.score, row.basicInfo.headcalcscore, row.basicInfo.head, row.basicInfo.headcount, row.basicInfo.havaas) + "'></div>";
}
}, {
targets: 1,
width: "10%",
render: function(data, type, row, meta) {
return "<span class='bold'>" + row.basicInfo.score + "</span>";
}
}, {
targets: 2,
width: "50%",
render: function(data, type, row, meta) {
var html = "<span style='font-size:16px;' class='bold'>" + row.name + "</span>";
html += "</br><span>" + row.basicInfo.job + "</span>";
if(row.basicInfo.nationInfo) {
html += " | <img style='width: 24px' title='" + row.basicInfo.nationInfo.name + "' src='" + row.basicInfo.nationInfo.icon + "'>";
}
if(row.basicInfo.leagueInfo) {
html += " | <img style='width: 24px' title='" + row.basicInfo.leagueInfo.name + "' src='" + row.basicInfo.leagueInfo.icon + "'>";
}
if(row.basicInfo.clubInfo) {
html += " | <img style='width: 24px' title='" + row.basicInfo.clubInfo.name + "' src='" + row.basicInfo.clubInfo.icon + "'>";
}
html += "</br><span>" + (row.basicInfo.programInfo.code == 'basic' ? '基本球员' : (row.basicInfo.programInfo.code == 'wc' ? '世界杯球员' : row.basicInfo.programInfo.name)) + "</span>";
return html;
}
}, {
targets: 3,
width: "15%",
render: function(data, type, row, meta) {
return "<span class='bold'>" + (parseInt(row.values[0]) + parseInt(row.values[1]) +
parseInt(row.values[2]) + parseInt(row.values[3]) + parseInt(row.values[4]) +
parseInt(row.values[5])) + "</span>";
}
}, {
targets: 4,
width: "15%",
render: function(data, type, row, meta) {
var value = 0;
for(var i = 0; i < row.capabilityValues.extend.length; i++) {
value += parseInt(row.capabilityValues.extend[i]);
}
return "<span class='bold'>" + value.toString() + "</span>";
}
}, {
targets: 5,
className: "hidden",
render: function(data, type, row, meta) {
return "<span class='bold'>" + row.values[0] + "</span>";
}
}, {
targets: 6,
className: "hidden",
render: function(data, type, row, meta) {
return "<span class='bold'>" + row.values[1] + "</span>";
}
}, {
targets: 7,
className: "hidden",
render: function(data, type, row, meta) {
return "<span class='bold'>" + row.values[2] + "</span>";
}
}, {
targets: 8,
className: "hidden",
render: function(data, type, row, meta) {
return "<span class='bold'>" + row.values[3] + "</span>";
}
}, {
targets: 9,
className: "hidden",
render: function(data, type, row, meta) {
return "<span class='bold'>" + row.values[4] + "</span>";
}
}, {
targets: 10,
className: "hidden",
render: function(data, type, row, meta) {
return "<span class='bold'>" + row.values[5] + "</span>";
}
}, {
targets: 11,
className: "hidden",
render: function(data, type, row, meta) {
return row.capabilityValues.extend[0];
}
}, {
targets: 12,
className: "hidden",
render: function(data, type, row, meta) {
return row.capabilityValues.extend[1];
}
}, {
targets: 13,
className: "hidden",
render: function(data, type, row, meta) {
return row.capabilityValues.extend[2];
}
}, {
targets: 14,
className: "hidden",
render: function(data, type, row, meta) {
return row.capabilityValues.extend[3];
}
}, {
targets: 15,
className: "hidden",
render: function(data, type, row, meta) {
return row.capabilityValues.extend[4];
}
}, {
targets: 16,
className: "hidden",
render: function(data, type, row, meta) {
return row.capabilityValues.extend[5];
}
}, {
targets: 17,
className: "hidden",
render: function(data, type, row, meta) {
return row.capabilityValues.extend[6];
}
}, {
targets: 18,
className: "hidden",
render: function(data, type, row, meta) {
return row.capabilityValues.extend[7];
}
}, {
targets: 19,
className: "hidden",
render: function(data, type, row, meta) {
return row.capabilityValues.extend[8];
}
}, {
targets: 20,
className: "hidden",
render: function(data, type, row, meta) {
return row.capabilityValues.extend[9];
}
}, {
targets: 21,
className: "hidden",
render: function(data, type, row, meta) {
return row.capabilityValues.extend[10];
}
}, {
targets: 22,
className: "hidden",
render: function(data, type, row, meta) {
return row.capabilityValues.extend[11];
}
}, {
targets: 23,
className: "hidden",
render: function(data, type, row, meta) {
return row.capabilityValues.extend[12];
}
}, {
targets: 24,
className: "hidden",
render: function(data, type, row, meta) {
return row.capabilityValues.extend[13];
}
}, {
targets: 25,
className: "hidden",
render: function(data, type, row, meta) {
return row.capabilityValues.extend[14];
}
}, {
targets: 26,
className: "hidden",
render: function(data, type, row, meta) {
return row.capabilityValues.extend[15];
}
}, {
targets: 27,
className: "hidden",
render: function(data, type, row, meta) {
return row.capabilityValues.extend[16];
}
}, {
targets: 28,
className: "hidden",
render: function(data, type, row, meta) {
return row.capabilityValues.extend[17];
}
}, {
targets: 29,
className: "hidden",
render: function(data, type, row, meta) {
return row.capabilityValues.extend[18];
}
}, {
targets: 30,
className: "hidden",
render: function(data, type, row, meta) {
return row.capabilityValues.extend[19];
}
}],
"bRetrieve": true,
"searching": false,
"lengthChange": false,
"pageLength": 10,
"deferRender": true,
"language": {
"lengthMenu": "显示 _MENU_ 条记录",
"loadingRecords": "请等待,数据正在加载中......",
"infoEmpty": "没有记录可以显示",
"emptyTable": "无可用数据",
"info": "",
"paginate": {
"first": "<<",
"last": ">>",
"next": ">",
"previous": "<"
}
}
});
setTimeout(function() {
$("#dataTables_paginate").parent().removeClass("col-md-7");
}, 5000)
}
function clearFilter() {
$("#name").val('');
$("#height").val('');
$("#foot").val('');
$("#scoreMin").val('');
$("#scoreMax").val('');
$("#nationSelect").multiselect("deselect", selectedNation);
selectedNation = "";
$("#raritySelect").multiselect("deselect", selectedRaritie);
selectedRaritie = "";
$("#jobSelect").multiselect("deselect", selectedJob);
selectedJob = "";
$("#leagueSelect").multiselect("deselect", selectedLeague);
selectedLeague = "";
$("#clubSelect").multiselect("deselect", selectedClub);
selectedClub = "";
$("#programSelect").multiselect("deselect", selectedProgram);
selectedProgram = "";
selectedProgramChild = "";
$("#programChildDiv").hide();
$("#skillSelect").multiselect("deselect", selectedSkill);
selectedSkill = "";
}<file_sep>/qingstorHandler.py
# -*- coding:utf-8 -*-
from qingstor.sdk.service.qingstor import QingStor
from qingstor.sdk.config import Config
import requests
import tempfile
# 初始化QingStor对象
config = Config('EBZAULNLJTMJJCZJWCRG', '02MLUQZA5wZPwTrOmVWUajPhNUSFI8c5VKu2Wf0u')
qingstor = QingStor(config)
# 获取bucket列表
# output = qingstor.list_buckets()
# print(output.status_code)
# print(output['buckets'])
# 获取指定bucket对象
bucket = qingstor.Bucket('fifa-helper-bucket', 'sh1a')
def getFileByKey(key):
resp = bucket.get_object(key)
with tempfile.NamedTemporaryFile() as f:
for chunk in resp.iter_content():
f.write(chunk)
return f
def getRespByKey(key):
resp = bucket.get_object(key)
return resp
# 上传本地文件,图片使用'rb'格式,文档使用'r'
# with open('1625_IC1.png', 'rb') as f:
# output = bucket.put_object(
# 'test-key', body=f
# )
# print(output.status_code)
# 上传网络文件,图片使用'rb'格式,文档使用'r'
# r = requests.get('http://img.wantuole.com/resources/backgrouds/VIP2.png')
# output = bucket.put_object(
# 'test-key-2.png', body=r
# )
# print('结果:', output.status_code)
# 获取bucket文件列表
# output = bucket.list_objects()
# print(output.status_code)
# print("文件列表:", output['keys'])
#
# resp = bucket.head_object('test-key-2.png')
# print("文件状态:", resp.status_code)
# 根据key获取具体文件
# resp = bucket.get_object('test-key-2.png')
# with tempfile.NamedTemporaryFile() as f:
# for chunk in resp.iter_content():
# f.write(chunk)
# 根据key删除文件
# resp = bucket.delete('test-key-2.png')
# print("删除状态:", resp.status_code)<file_sep>/esHandler.py
# -*- coding: utf-8 -*-
import requests
import json
from elasticsearch import Elasticsearch
"""球员列表url"""
base_list_url = 'http://api.wantuole.com/api/fifa/list2?1=1&mi=%d&ma=%d'
base_list_url_with_job = 'http://api.wantuole.com/api/fifa/list2?1=1&j=%s&mi=%d&ma=%d'
"""国家列表url"""
nation_list_url = 'http://api.wantuole.com/api/nation/list'
"""联赛列表url"""
league_list_url = 'http://api.wantuole.com/api/league/list'
"""俱乐部列表url"""
club_list_url = 'http://api.wantuole.com/api/club/list?lcode='
"""天赋列表url"""
skill_list_url = 'http://api.wantuole.com/api/skill/list?gameCode=fifamobile'
"""天赋等级提升(单位:百分比)url"""
skill_level_capability_url = 'http://api.wantuole.com/api/levelup/list?gameCode=fifamobile&type=2&level=0&code='
"""位置等级提升url"""
job_level_capability_url = 'http://api.wantuole.com/api/levelup/list?gameCode=fifamobile&type=1&level=60&code='
"""活动列表url"""
program_list_url = 'http://api.wantuole.com/api/program/list?gameCode=fifamobile'
"""球员位置: 前锋 左边锋 右边锋 左内锋 中锋 右内锋 前腰 左前卫 中前卫 右前卫 后腰 左翼卫 左后卫 中后卫 右后卫 右翼卫 门将"""
job_list = ["ST", "LW", "RW", "LF", "CF", "RF", "CAM", "LM", "CM", "RM", "CDM", "LWB", "LB", "CB", "RB", "RWB", "GK"]
"""es连接"""
#TODO 设置最大连接数
es = Elasticsearch(['http://127.0.0.1:9200'])
"""球员索引"""
fifa_player_index = "fifa-player-index"
"""球员type"""
fifa_player_type = "fifa-player-type"
"""国家索引"""
fifa_nation_index = "fifa-nation-index"
"""国家type"""
fifa_nation_type = "fifa-nation-type"
"""联赛索引"""
fifa_league_index = "fifa-league-index"
"""联赛type"""
fifa_league_type = "fifa-league-type"
"""俱乐部索引"""
fifa_club_index = "fifa-club-index"
"""俱乐部type"""
fifa_club_type = "fifa-club-type"
"""天赋索引"""
fifa_skill_index = "fifa-skill-index"
"""天赋type"""
fifa_skill_type = "fifa-skill-type"
"""活动索引"""
fifa_program_index = "fifa-program-index"
"""活动type"""
fifa_program_type = "fifa-program-type"
"""天赋等级提升索引"""
fifa_skill_level_capability_index = 'fifa_skill_level_capability_index'
"""天赋等级提升type"""
fifa_skill_level_capability_type = 'fifa_skill_level_capability_type'
"""位置等级提升索引"""
fifa_job_level_capability_index = 'fifa_job_level_capability_index'
"""位置等级提升type"""
fifa_job_level_capability_type = 'fifa_job_level_capability_type'
"""默认取回200条数据"""
default_search_body = {"from": 0, "size": 200}
"""
从玩脱了爬取球员数据
"""
def downloadPlayersByURL(url):
resp = requests.get(url)
data = json.loads(resp.text)
num = len(data['data'])
print(url, ' ===》 数量:', num)
p = {}
for player in data['data']:
setValue(p, player, 'code', 'code', None)
setValue(p, player, 'name', 'name', None)
setValue(p, player, 'rarityValue', 'rarityValue', None)
setValue(p, player, 'rarity', 'rarity', None)
"""basicInfo"""
setValue(p, player, 'no', 'basicInfo', 'no')
setValue(p, player, 'enname', 'basicInfo', 'enname')
setValue(p, player, 'score', 'basicInfo', 'score')
setValue(p, player, 'jobCode', 'basicInfo', 'jobCode')
setValue(p, player, 'job', 'basicInfo', 'job')
p['nation'] = player['basicInfo']['nationInfo']['name']
p['league'] = player['basicInfo']['leagueInfo']['name']
p['club'] = player['basicInfo']['clubInfo']['name']
p['program'] = player['basicInfo']['programInfo']['name']
setValue(p, player, 'bg', 'basicInfo', 'bg')
setValue(p, player, 'programicon', 'basicInfo', 'programicon')
setValue(p, player, 'head', 'basicInfo', 'head')
setValue(p, player, 'headcount', 'basicInfo', 'headcount')
setValue(p, player, 'headcalcscore', 'basicInfo', 'headcalcscore')
setValue(p, player, 'havaas', 'basicInfo', 'havaas')
setValue(p, player, 'height', 'basicInfo', 'height')
setValue(p, player, 'foot', 'basicInfo', 'foot')
setValue(p, player, 'basicdownstatus', 'basicInfo', 'basicdownstatus')
setValue(p, player, 'traits', 'basicInfo', 'traits')
setValue(p, player, 'downstatus', 'basicInfo', 'downstatus')
"""capabilityValues"""
setValue(p, player, 'capabilityValues', 'capabilityValues', 'extend')
"""skills"""
setValue(p, player, 'skill_code', 'skills', 'code')
setValue(p, player, 'skill_name', 'skills', 'name')
print(p)
#存入es
es.index(index=fifa_player_index, doc_type=fifa_player_type, id=p['code'], body=p)
player['basicInfo']['nationInfo']['name']
background_pic_filename = getBackground(player['basicInfo']['programInfo']['name'], player['rarityValue'], player['basicInfo']['score'],
player['basicInfo']['programInfo']['havebackground'],player['basicInfo']['bg'])
background_url = getBackgroundURL(player['basicInfo']['programInfo']['name'], background_pic_filename)
head_pic_filename = getHead(player['basicInfo']['programInfo']['name'], player['basicInfo']['no'], player['basicInfo']['score'],
player['basicInfo']['headcalcscore'], player['basicInfo']['head'],
player['basicInfo']['headcount'], player['basicInfo']['havaas'])
head_url = getHeadURL(player['basicInfo']['programInfo']['name'], head_pic_filename)
print('背景:', background_pic_filename, ' -> ', background_url)
print('头像:', head_pic_filename, ' -> ', head_url)
def setValue(p1, p2, p1_colume, p2_colume1, p2_colume2):
if p2_colume2 is None:
p1[p1_colume] = p2[p2_colume1]
elif p2_colume2 in p2[p2_colume1]:
p1[p1_colume] = p2[p2_colume1][p2_colume2]
else:
p1[p1_colume] = None
"""
初始化球员索引
"""
def initPlayerIndex(min_score, max_score, split_score):
# 删除原有索引
es.indices.delete(index=fifa_player_index, ignore=[400, 404])
# 重新创建索引
es.indices.create(index=fifa_player_index, ignore=400)
# 启用fielddata,关闭analyzed
body = {
"properties": {
"score": {
"type": "text",
"fielddata": True
}, "nation": {
"type": "keyword"
}, "league": {
"type": "keyword"
}, "club": {
"type": "keyword"
}, "job": {
"type": "keyword"
}, "skill": {
"type": "keyword"
}, "program": {
"type": "keyword"
}
}
}
es.indices.put_mapping(index=fifa_player_index, doc_type=fifa_player_type, body=body)
# 抓取球员数据
downloadPlayers(min_score, max_score, split_score)
"""
爬取球员数据
"""
def downloadPlayers(min_score, max_score, split_score):
i = max_score
while i >= min_score:
if i > split_score:
downloadPlayersByURL(base_list_url%(i,i))
else:
for job in job_list:
downloadPlayersByURL(base_list_url_with_job % (job, i, i))
i = i-1
"""
初始化国家索引
"""
def initNationIndex():
# 删除原有索引
es.indices.delete(index=fifa_nation_index, ignore=[400, 404])
# 重新创建索引
es.indices.create(index=fifa_nation_index, ignore=400)
# 爬取国家列表
print(nation_list_url)
resp = requests.get(nation_list_url)
data = json.loads(resp.text)
print(data)
n = {}
for nation in data['data']:
setValue(n, nation, 'code', 'code', None)
setValue(n, nation, 'icon', 'icon', None)
setValue(n, nation, 'chname', 'chname', None)
setValue(n, nation, 'enname', 'enname', None)
print(n)
es.index(index=fifa_nation_index, doc_type=fifa_nation_type, id=n['code'], body=n)
"""
search国家
"""
def searchNation():
resp = es.search(index=fifa_nation_index, doc_type=fifa_nation_type, body=default_search_body)
data = tranferDictWithUTF8(resp["hits"])
print(data)
return data
"""
初始化联赛/俱乐部索引
"""
def initLeagueAndClubIndex():
# 删除原有索引
es.indices.delete(index=fifa_league_index, ignore=[400, 404])
es.indices.delete(index=fifa_club_index, ignore=[400, 404])
# 重新创建索引
es.indices.create(index=fifa_league_index, ignore=400)
es.indices.create(index=fifa_club_index, ignore=400)
# 关闭俱乐部analyzed,以便可以按照联赛全匹配
body = {
"properties": {
"leagueName": {
"type": "keyword"
}
}
}
es.indices.put_mapping(index=fifa_club_index, doc_type=fifa_club_type, body=body)
# 爬取联赛列表
print(league_list_url)
resp = requests.get(league_list_url)
data = json.loads(resp.text)
print(data)
n = {}
for league in data['data']:
setValue(n, league, 'code', 'code', None)
setValue(n, league, 'icon', 'icon', None)
setValue(n, league, 'chname', 'chname', None)
setValue(n, league, 'enname', 'enname', None)
print(n)
es.index(index=fifa_league_index, doc_type=fifa_league_type, id=n['code'], body=n)
# 爬取俱乐部列表
print(club_list_url)
resp2 = requests.get(club_list_url + n['code'])
data2 = json.loads(resp2.text)
print(data2)
c = {'leagueName': n['chname']}
for club in data2['data']:
setValue(c, club, 'code', 'code', None)
setValue(c, club, 'icon', 'icon', None)
setValue(c, club, 'chname', 'chname', None)
setValue(c, club, 'enname', 'enname', None)
print(c)
es.index(index=fifa_club_index, doc_type=fifa_club_type, id=c['code'], body=c)
"""
search联赛
"""
def searchLeague():
resp = es.search(index=fifa_league_index, doc_type=fifa_league_type, body=default_search_body)
data = tranferDictWithUTF8(resp["hits"])
print(data)
return data
"""
search俱乐部
"""
def searchClub(leagueName):
body = {"from": 0, "size":500}
if leagueName is not None:
body['query'] = {'term': {'leagueName': leagueName}}
resp = es.search(index=fifa_club_index, doc_type=fifa_club_type, body=body)
data = tranferDictWithUTF8(resp["hits"])
print(data)
return data
"""
初始化天赋索引
"""
def initSkillIndex():
# 删除原有索引
es.indices.delete(index=fifa_skill_index, ignore=[400, 404])
es.indices.delete(index=fifa_skill_level_capability_index, ignore=[400, 404])
# 重新创建索引
es.indices.create(index=fifa_skill_index, ignore=400)
es.indices.create(index=fifa_skill_level_capability_index, ignore=400)
# 取消analyzed,以便全匹配搜索
# body = {'properties': {'code': {'type': 'keyword'}}}
# es.indices.put_mapping(index=fifa_skill_level_capability_index, doc_type=fifa_skill_level_capability_type, body=body)
# 爬取天赋列表
print(skill_list_url)
resp = requests.get(skill_list_url)
data = json.loads(resp.text)
print(data)
n = {}
for skill in data['data']:
# 天赋
setValue(n, skill, 'code', 'code', None)
setValue(n, skill, 'name', 'name', None)
print(n)
es.index(index=fifa_skill_index, doc_type=fifa_skill_type, id=n['code'], body=n)
# 天赋等级提升
url2 = skill_level_capability_url+skill['code'];
resp2 = requests.get(url2)
data2 = json.loads(resp2.text)
s = {'code': skill['code'], 'upData': data2['data']}
es.index(index=fifa_skill_level_capability_index, doc_type=fifa_skill_level_capability_type, id=skill['code'], body=s)
"""
search天赋
"""
def searchSkill():
resp = es.search(index=fifa_skill_index, doc_type=fifa_skill_type, body=default_search_body)
data = tranferDictWithUTF8(resp["hits"])
print(data)
return data
"""
search天赋等级提升
"""
def searchSkillLevelCapability(code):
# body = {'query': {'term': {'code': code}}}
# resp = es.search(index=fifa_skill_level_capability_index, doc_type=fifa_skill_level_capability_type, body=body)
resp = es.get(index=fifa_skill_level_capability_index, doc_type=fifa_skill_level_capability_type, id=code)
data = tranferDictWithUTF8(resp["_source"])
print(data)
return data
"""
初始化活动索引
"""
def initProgramIndex():
# 删除原有索引
es.indices.delete(index=fifa_program_index, ignore=[400, 404])
# 重新创建索引
es.indices.create(index=fifa_program_index, ignore=400)
# 爬取活动列表
print(program_list_url)
resp = requests.get(program_list_url)
data = json.loads(resp.text)
print(data)
n = {}
for program in data['data']:
if program['isShow'] == '1':
setValue(n, program, 'code', 'code', None)
setValue(n, program, 'icon', 'icon', None)
setValue(n, program, 'chname', 'chname', None)
setValue(n, program, 'enname', 'enname', None)
print(n)
es.index(index=fifa_program_index, doc_type=fifa_program_type, id=n['code'], body=n)
"""
search活动
"""
def searchProgram():
resp = es.search(index=fifa_program_index, doc_type=fifa_program_type, body=default_search_body)
data = tranferDictWithUTF8(resp["hits"])
print(data)
return data
"""
初始化位置等级提升索引
"""
def initJobLevelCapabilityIndex():
# 删除原有索引
es.indices.delete(index=fifa_job_level_capability_index, ignore=[400, 404])
# 重新创建索引
es.indices.create(index=fifa_job_level_capability_index, ignore=400)
# 取消analyzed,以便全匹配搜索
# body = {'properties': {'code': {'type': 'keyword'}}}
# es.indices.put_mapping(index=fifa_job_level_capability_index, doc_type=fifa_job_level_capability_type, body=body)
# 爬取位置等级提升数据
for job in job_list:
url = job_level_capability_url+job;
print(url)
resp = requests.get(url)
data = json.loads(resp.text)
j = {'code': job, 'upData': data['data']}
print(j)
es.index(index=fifa_job_level_capability_index, doc_type=fifa_job_level_capability_type, id=j['code'], body=j)
"""
search位置等级提升
"""
def searchJobLevelCapability(code):
# body = {'query': {'term': {'code': code}}}
# resp = es.search(index=fifa_job_level_capability_index, doc_type=fifa_job_level_capability_type, body=body)
resp = es.get(index=fifa_job_level_capability_index, doc_type=fifa_job_level_capability_type, id=code)
data = tranferDictWithUTF8(resp["_source"])
print(data)
return data
"""
count球员
"""
def countPlayers():
resp = es.count(index=fifa_player_index, doc_type=fifa_player_type)
return str(resp['count'])
"""
count所有信息,包括球员/联赛/俱乐部/天赋/活动
"""
def countAll():
player_count = es.count(index=fifa_player_index, doc_type=fifa_player_type)['count']
league_count = es.count(index=fifa_league_index, doc_type=fifa_league_type)['count']
club_count = es.count(index=fifa_club_index, doc_type=fifa_club_type)['count']
skill_count = es.count(index=fifa_skill_index, doc_type=fifa_skill_type)['count']
program_count = es.count(index=fifa_program_index, doc_type=fifa_program_type)['count']
skill_updata_count = es.count(index=fifa_skill_level_capability_index, doc_type=fifa_skill_level_capability_type)['count']
job_updata_count = es.count(index=fifa_job_level_capability_index, doc_type=fifa_job_level_capability_type)['count']
result = {
'player_count': player_count,
'league_count': league_count,
'club_count': club_count,
'skill_count': skill_count,
'program_count': program_count,
'skill_updata_count': skill_updata_count,
'job_updata_count': job_updata_count
}
return tranferDictWithUTF8(result)
"""
search球员
"""
def searchPlayers(min_score, max_score, name, nation, league, club, job, skill, program):
body = {
"from": 0, "size": 100,
"query": {
"bool": {
"must": [
]
}
},
"sort": {
"score": {
"order": "desc"
}
}
}
if min_score is not None or max_score is not None:
score_range = {"range": {"score": {}}}
if min_score is not None:
score_range["range"]["score"]["gte"] = int(min_score)
if max_score is not None:
score_range["range"]["score"]["lte"] = int(max_score)
body["query"]["bool"]["must"].append(score_range)
if name is not None:
name_match = {"match": {"name": name}}
body["query"]["bool"]["must"].append(name_match)
if nation is not None:
nation_match = {"term": {"nation": nation}}
body["query"]["bool"]["must"].append(nation_match)
if league is not None:
league_match = {"term": {"league": league}}
body["query"]["bool"]["must"].append(league_match)
if club is not None:
club_match = {"term": {"club": club}}
body["query"]["bool"]["must"].append(club_match)
if job is not None:
job_match = {"term": {"job": job}}
body["query"]["bool"]["must"].append(job_match)
if skill is not None:
skill_match = {"term": {"skill": skill}}
body["query"]["bool"]["must"].append(skill_match)
if program is not None:
program_match = {"term": {"program": program}}
body["query"]["bool"]["must"].append(program_match)
print(body)
resp = es.search(index=fifa_player_index, doc_type=fifa_player_type, body=body)
result = tranferDictWithUTF8(resp["hits"])
print(result)
return result
"""
将字典转为UTF8字符串
"""
def tranferDictWithUTF8(dict):
result = json.dumps(dict, ensure_ascii=False).encode('utf-8')
# print(type(result))
return result
# ==================================================获取球员照片=======================================================
"""
获取背景图片URL
"""
def getBackgroundURL(pn, picFilename):
imgUrl = "http://img.wantuole.com/resources/backgrouds/"
return imgUrl + picFilename
def getBackground(pn, r, score, hb, bg):
num = 0
imgName = ""
if int(hb) > 0:
num = int((int(score) - 70) / 10)
if bg == "CGER" or bg == 'SC' or bg == 'TC' or bg == 'T' or bg == 'TDYX' or pn.find('剧情精英球员') >= 0 or bg == 'ZCBase':
num = int((int(score) - 50) / 10)
elif bg == 'JLBZ':
num = int((int(score) - 60) / 10)
if pn == '世界杯球员':
if num > 4:
num = 4
imgName = bg + str(num) + ".png"
else:
num = int((int(score) - 50) / 10);
if bg != "BASIC":
imgName = bg + str(num) + ".png"
else:
r_int = int(r)
if r_int == 1:
imgName = ""
elif r_int == 2:
imgName = "MR"
elif r_int == 3:
imgName = "ER"
elif r_int == 4:
imgName = "GR"
elif r_int == 5:
imgName = "SR"
elif r_int == 6:
imgName = "BR"
imgName = imgName + str(num) + ".png"
return imgName
"""
获取球员照片URL
"""
def getHeadURL(pn, picFilename):
imgUrl = "http://img.wantuole.com/FIFA足球世界/players/"
if pn == '世界杯球员':
imgUrl = "http://img.wantuole.com/FIFA足球世界/wcplayers/"
return imgUrl + picFilename
def getHead(pn, no, score, calcscore, head, count, haveas):
imgUrl = "http://img.wantuole.com/FIFA足球世界/players/"
if pn == '世界杯球员':
imgUrl = "http://img.wantuole.com/FIFA足球世界/wcplayers/"
num = 0
imgName = ""
if haveas != "" and int(haveas) == 1:
if int(score) >= 80:
if calcscore is not None:
imgName = no + "_AS" + calcscore + ".png"
else:
imgName = no + "_AS.png"
else:
imgName = no + ".png"
else:
if head == "TOTW":
#TODO 可能有bug
if score is not None and score != "":
imgName = no + "_" + head + calcscore + ".png"
else:
imgName = no + "_" + head + ".png"
else:
if count != "" and int(count) > 1:
num = int((int(score) - int(calcscore)) / 10)
if head == "IC" or head == "PI":
if num == 0:
num = 1
if num > int(count):
num = int(count)
imgName = no + "_" + head + str(num) + ".png"
else:
if head is not None and head != "":
if int(score) >= 80:
imgName = no + "_" + head + ".png"
if head == 'MM':
if score >= 100:
imgName = no + "_L" + head + ".png"
elif head == 'LVL':
if score >= 100:
imgName = no + "_LVLM.png"
else:
if calcscore == -1:
imgName = no + "_" + head + ".png"
else:
imgName = no + ".png"
else:
imgName = no + ".png"
return imgName | dbb01c42668dc76d4513c4e5356fdf5e0f9dc653 | [
"JavaScript",
"Python"
] | 7 | Python | melo1024/flaskWeb | 95813db2e4ff5dff66a9cfe793ec8ef06ae3ea67 | 4cc9340fd442fa4ff5f0336685e2a55b17893021 | |
refs/heads/master | <repo_name>nghiavtn12/LearnSomethingEveryday<file_sep>/README.md
# LearnSomethingEveryday
Learn More Do More Play More
<file_sep>/login-getlistbyjs/common.js
// calendar
$(function () {
var $date = $('.calendar');
var options = {
show: function (e) {},
hide: function (e) {},
pick: function (e) {},
format: 'dd/mm/yyyy',
autoHide: true,
zIndex: 9999
};
$date.on({
'show.datepicker': function (e) {},
'hide.datepicker': function (e) {},
'pick.datepicker': function (e) {}
}).datepicker(options);
})<file_sep>/ReactJS/react02h/src/Component/convertST.js
const convertDate = (date) => {
var dateStd = date
.split(" ")[0]
.split("-")
.reverse()
.join("/");
return dateStd;
}
const converTransferType = (type) => {
var typeVi = (type === 'move_sale' ? 'Chuyển bán' : 'Chuyển liên khu vực');
return typeVi;
}
export {
convertDate,
converTransferType,
}
<file_sep>/login-getlistbyjs/getlist.js
$(function () {
if (
localStorage.getItem("token") === null ||
localStorage.getItem("token") === "undefined"
) {
alert("Vui lòng đăng nhập");
window.location.href = "index.html";
} else {
$("#username").text(localStorage.getItem("username"));
getDataFillter();
$.ajax({
url: "http://103.68.68.142:8000/wfs/co/filter",
headers: {
Authorization: localStorage.getItem("token"),
"Content-Type": "application/x-www-form-urlencoded"
},
dataType: "json",
data: {},
success: function (result) {
var a = result.data.seller;
var b = '<option value = "" >Tất cả</option> ';
a.forEach(element => {
b += "<option value='" + element.id + "'>";
b += element.name;
b += "</option>";
});
$("#seller").html(b);
}
});
$.ajax({
url: "http://192.168.3.11:8000/wfs/ir/get-filters?location_base=1&location_transfer=1",
headers: {
Authorization: localStorage.getItem("token"),
"Content-Type": "application/x-www-form-urlencoded"
},
dataType: "json",
data: {},
success: function (result) {
var a = result.location;
var b = '<option value = " ">Tất cả</option> ';
a.forEach(element => {
b += "<option value='" + element.id + "'>";
b += element.name;
b += "</option>";
});
$(".warehouse").html(b);
}
});
}
});
function getLogout() {
localStorage.removeItem("token");
localStorage.removeItem("username");
window.location.href = "index.html";
}
function convertState(item) {
var state = " ";
switch (item) {
case "draft":
state = "Chờ luân chuyển";
break;
case "tr_picking":
state = "Lấy hàng";
break;
case "tr_picked":
state = "Đang luân chuyển";
break;
case "done":
state = "Xong";
break;
case "cancel":
state = "Hủy";
break;
}
return state;
}
function convertDate(date) {
var x = date
.split(" ")[0]
.split("-")
.reverse()
.join("-");
return x;
}
function checkdate() {
console.log($("#fromdate").val());
}
function checkFillter() {
var a = {
"code": $("#code").val(),
"seller": $("#seller").val(),
"from_date": $("#fromdate").val().split("/").reverse().join("-"),
"to_date": $("#todate").val().split("/").reverse().join("-"),
"location": $("#warehouse").val(),
"location_dest": $("#warehouse_dest").val(),
"state": $("#state").val(),
"transfer_type": $("#type").val(),
"limit": $("#limit").val()
};
for (x in a) {
if (a[x] == null || a[x] == undefined || a[x] == " " || a[x] == "") {
delete a[x]
}
}
console.log(a)
getDataFillter(a)
}
function changePage(page) {
console.log($(page).attr("data-id"))
var cp = {"page": $(page).attr("data-id")}
getDataFillter(cp)
}
function getDataFillter(data) {
$.ajax({
url: "http://192.168.3.11:8000/wfs/transfer/list?",
headers: {
Authorization: localStorage.getItem("token"),
"Content-Type": "application/x-www-form-urlencoded"
},
dataType: "json",
data: data,
success: function (result) {
var a = result.data.records;
var b = " ";
var TotalPage = Math.ceil(result.data.total / $("#limit").val());
var liPage = "";
for(i=1;i<TotalPage+1;i++)
{
liPage+="<li onclick='changePage(this)' data-id=" + i +"><a>"+i+"</a></li>"
}
$("#tbpage").html(liPage)
a.forEach(element => {
b += "<tr><td>";
b += element.code;
b += "</td><td>";
b += element.seller;
b += "</td><td>";
b += convertDate(element.create_date);
b += "</td><td>";
b += element.warehouse;
b += "</td><td>";
b += element.warehouse_dest;
b += "</td><td>";
b +=
element.transfer_type === "move_sale" ?
"Chuyển bán" :
"Chuyển liên khu vực";
b += "</td><td>";
b += element.product_qty;
b += "</td><td>";
b += convertState(element.state);
b += "</td></tr>";
});
$("#trth").html(b);
},
error: function (request, status, error) {
alert("Có lỗi trong quá trình thực thi. Vui lòng đăng nhập lại");
}
});
}
<file_sep>/JS/popupAuto/popup.js
isLoaded = () => {
setTimeout(()=>{$("#popup").css("display","none");
$("#main").css("display","block");},3000);
}
isLoaded();<file_sep>/ReactJS/react02h/src/Component/LoginJS.js
import React, { Component } from 'react';
import { Redirect } from 'react-router-dom';
import axios from 'axios';
import './LoginJS.scss'
class Login extends Component {
constructor(props) {
super(props);
this.state = {
isLogin: false,
}
}
onBtnClick = () => {
const email = document.getElementById('username').value;
const password = document.getElementById('password').value;
const formData = new FormData();
formData.set('email',email);
formData.set('password',<PASSWORD>);
axios.post('https://master-wfs.agilsun.com/wfs/auth/token', formData)
.then((res) => {
console.log(res.data);
localStorage.setItem('token',res.data.token);
this.setState({isLogin: true})
})
.catch(function (error) {
this.setState({
error: true
});
console.log(error);
})
}
handleKeyPress = (e = '') => {
if (e.key === 'Enter') {
this.onBtnClick();
}
}
render() {
if(this.state.isLogin) {
return <Redirect to="/list" />
}
return (
<div className="LoginForm">
<input type="text" name="username" id="username" placeholder="Username" onKeyPress={this.handleKeyPress}/><br/>
<input type="password" name="password" id="password" placeholder="<PASSWORD>" onKeyPress={this.handleKeyPress}/><br/>
<button type="button" onClick={this.onBtnClick}>Login</button>
</div>
)
}
}
export default Login
<file_sep>/ReactJS/movie-center-web/src/components/menu/menu.js
import React, { Component } from 'react';
import logo from '../../asset/img/logo.jpg';
import './menu.css';
class Menu extends Component {
render() {
return (
<div className='top-menu'>
<img src = {logo} alt="logo"/>
<input type="text" placeholder = "Tìm kiếm"/>
<a src="#" data-toggle="modal" data-target="#myModal">Đăng nhập/đăng ký</a>
<button type="button" class="btn btn-info btn-lg" data-toggle="modal" data-target="#myModal">Open Modal</button>
<div id="myModal" class="modal fade" role="dialog">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal">×</button>
<h4 class="modal-title">Modal Header</h4>
</div>
<div class="modal-body">
<p>Some text in the modal.</p>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
</div>
)
}
}
export default Menu;<file_sep>/JS/cal/cal.js
var valu = '',result = '';
document.getElementById('screenCal').value = 0;
resetValue = () => {
this.valu = '';
document.getElementById('screenCal').value = '0';
}
getValueInput = (v) => {
valu += v.value;
document.getElementById('screenCal').value = valu;
}
getCalculate = (u) => {
var a = document.getElementById('screenCal').value;
a += " " + u.value + " " ;
valu = a;
document.getElementById('screenCal').value = a;
}
getCalculating = (arr,i) => {
var res;
switch (cal[i]) {
case "+":
res = parseFloat(cal[i-1]) + parseFloat(cal[i+1]);
break;
case "-":
res = parseFloat(cal[i-1]) - parseFloat(cal[i+1]);
break;
case "*":
res = parseFloat(cal[i-1]) * parseFloat(cal[i+1]);
break;
default:
if ( parseFloat(cal[i+1]) === 0 )
{
return alert("Không thể chia cho 0");
}
res = parseFloat(cal[i-1]) / parseFloat(cal[i+1]);
}
console.log(typeof(res));
return res;
}
excCal = () => {
var res,cal = document.getElementById('screenCal').value;
console.log(cal);
if(cal.length < 3) {
return;
}
cal = cal.split(' ');
console.log(cal,cal.length);
for (var i = 0; i < cal.length; i++) {
if(parseFloat(cal[i]) == NaN)
{
console.log('dzo');
res += getCalculating(cal,i);
}
}
document.getElementById('screenCal').value = res;
}
<file_sep>/JS/compare/compare.js
const a = [],b=[];
let i = 0;
let j = 0;
while(i < 10) {
a[i] = Math.floor((Math.random() * 100) + 1);
i++;
};
while(j < 10) {
b[j] = Math.floor((Math.random() * 100) + 1);
j++;
};
compare = (a,b) => {
console.log(a,b);
let res = [0,0];
for (var x = 0; x<10 ; x++) {
console.log(res[0],res[1]);
if(a[x]>b[x]) {
res[0]++;
}
else if (a[x]<b[x])
{
res[1]++;
}
}
console.log(res);
return res;
};
compare(a,b); | 66d24d07ccf585529ded36f6b2e9635ce5984046 | [
"Markdown",
"JavaScript"
] | 9 | Markdown | nghiavtn12/LearnSomethingEveryday | cf6a2ca591c35ec7c1ce51638f7dba004010c0e3 | 7df27bb46d373fb5e8ac4d8cff0c9eefdde09179 | |
refs/heads/master | <repo_name>brandedux/atm-sim<file_sep>/The-ATM-Machine/Migrations/20180127122839_CreateDB.cs
using Microsoft.EntityFrameworkCore.Migrations;
using System;
using System.Collections.Generic;
namespace TheATMMachine.Migrations
{
public partial class CreateDB : Migration
{
protected override void Up(MigrationBuilder migrationBuilder)
{
migrationBuilder.CreateTable(
name: "Accounts",
columns: table => new
{
AccountId = table.Column<int>(nullable: false)
.Annotation("Sqlite:Autoincrement", true),
BankNumber = table.Column<int>(nullable: false),
Money = table.Column<int>(nullable: false),
Pincode = table.Column<int>(maxLength: 4, nullable: false)
},
constraints: table =>
{
table.PrimaryKey("PK_Accounts", x => x.AccountId);
});
migrationBuilder.CreateTable(
name: "Users",
columns: table => new
{
UserId = table.Column<int>(nullable: false)
.Annotation("Sqlite:Autoincrement", true),
AccountId = table.Column<int>(nullable: false),
Name = table.Column<string>(nullable: true),
Surname = table.Column<string>(nullable: true)
},
constraints: table =>
{
table.PrimaryKey("PK_Users", x => x.UserId);
table.ForeignKey(
name: "FK_Users_Accounts_AccountId",
column: x => x.AccountId,
principalTable: "Accounts",
principalColumn: "AccountId",
onDelete: ReferentialAction.Cascade);
});
migrationBuilder.CreateIndex(
name: "IX_Users_AccountId",
table: "Users",
column: "AccountId",
unique: true);
}
protected override void Down(MigrationBuilder migrationBuilder)
{
migrationBuilder.DropTable(
name: "Users");
migrationBuilder.DropTable(
name: "Accounts");
}
}
}
<file_sep>/The-ATM-Machine/Machine/Interface.cs
using System;
using The_ATM_Machine.Queries;
using System.Threading;
namespace The_ATM_Machine {
/// <summary>
/// The ATM
/// </summary>
public class Machine {
QueryAccess queryAccess = new QueryAccess();
/// <summary>
/// Menu when the user is not logged in, shows the login, create and exit options
/// </summary>
public void StartUpMenuOptions() {
while (true) {
Thread.Sleep(2000);
Console.Clear();
Console.WriteLine("Welcome to the ATM \n Select one of the following options:");
Console.WriteLine("Login: 1 \n Create a bank account: 2 \n Exit: 3");
var answer = Console.ReadLine();
switch (answer.ToLower()) {
case ("1"):
this.Login();
return;
case ("2"):
this.CreateBankAccount();
break;
case ("3"):
Environment.Exit(0);
return;
default:
Console.WriteLine("Error: choose a valid menu option");
break;
}
}
}
/// <summary>
/// Menu when the user is logged in, shows the main options
/// </summary>
public void MainMenuOptions() {
while (true) {
Thread.Sleep(2000);
Console.Clear();
Console.WriteLine("Main Menu \n Welcome {0}", LoggedInUser.Name);
Console.WriteLine("Draw money: 1 \n Deposit: 2 \n Transfer money: 3 \n Change Pincode: 4 \n Current balance: 5 \n Exit: 6");
var answer = Console.ReadLine();
switch (answer.ToLower()) {
case ("1"):
this.Withdraw();
break;
case ("2"):
this.Deposit();
break;
case ("3"):
this.Transfer();
break;
case ("4"):
this.ChangePincode();
break;
case ("5"):
this.SeeBalance();
break;
case ("6"):
Environment.Exit(0);
break;
default:
Console.WriteLine("Error: choose a valid menu option");
break;
}
}
}
/// <summary>
/// This menu shows when the user enters invalid data
/// </summary>
/// <param name="backPredicate">the the method to call when the user chooses the back option</param>
/// <param name="retryPredicate">the the method to call when the user chooses the retry option</param>
private void BackRetryExit(Action backPredicate, Action retryPredicate) {
Console.WriteLine("Retry: 1 \n Back: 2 \n Exit: 3");
while (true) {
var answer = Console.ReadLine();
switch (answer.ToLower()) {
case ("1"):
retryPredicate();
return;
case ("2"):
backPredicate();
return;
case ("3"):
Environment.Exit(0);
return;
default:
Console.WriteLine("Error: select a valid menu option");
break;
}
}
}
/// <summary>
/// The login menu
/// </summary>
private void Login() {
Console.WriteLine("Login");
Console.WriteLine("Enter your bank account number");
var givenBanknumber = InputHandling.CheckNumber();
if (queryAccess.accountTable.CheckIfBankNumberExists(givenBanknumber)) {
var givenPincode = this.PincodeConfirmation("Enter your pincode");
if(queryAccess.accountTable.IsPincodeCorrect(givenBanknumber, givenPincode)) {
Console.WriteLine("Successfully logged in");
var loggedInUser = queryAccess.user.GetUserInformation(givenBanknumber);
LoggedInUser.LogInUser(loggedInUser.Item1, loggedInUser.Item2);
return;
}
Console.WriteLine("Error: The pincode is not correct");
}
else {
Console.WriteLine("Error: the bank account number has not been found");
}
this.BackRetryExit(() => this.StartUpMenuOptions(), () => this.Login());
}
/// <summary>
/// Create bank acount menu
/// </summary>
private void CreateBankAccount() {
Console.WriteLine("Create Bank Account");
Console.WriteLine("Type your name");
var name = Console.ReadLine();
Console.WriteLine("Type your surname");
var surname = Console.ReadLine();
Console.WriteLine("Type the bank account number that you would like to have");
var bankNumber = this.CheckIfBankNumberExists(InputHandling.CheckNumber());
var pincode = PincodeConfirmation("Type the pincode you would like to have");
queryAccess.accountTable.AddAccount(new Account() { BankNumber = bankNumber, Money = 0, Pincode = pincode });
queryAccess.user.AddUser(new User() { Name = name, Surname = surname, AccountId = queryAccess.accountTable.GetAccountId(bankNumber) });
Console.WriteLine("Registered \n you can login now");
}
/// <summary>
/// Used for the create bank account menu
/// </summary>
/// <param name="bankNumber">the banknumber that the user entered</param>
/// <returns></returns>
private int CheckIfBankNumberExists(int bankNumber) {
while (true) {
var doesBankAccountExist = queryAccess.accountTable.CheckIfBankNumberExists(bankNumber);
if (doesBankAccountExist) {
Console.WriteLine("Bank number already exists, try again:");
bankNumber = InputHandling.CheckNumber();
}
else {
return bankNumber;
}
}
}
/// <summary>
/// Checks if two inserted pincodes match and are correct
/// </summary>
/// <param name="message">message to display when the pincode confirmation screen is shown</param>
/// <returns></returns>
private int PincodeConfirmation(string message) {
while (true) {
Console.WriteLine(message);
var firstPincode = InputHandling.CheckNumber();
Console.WriteLine("Type your pincode again");
var secondPincode = InputHandling.CheckNumber();
if(firstPincode.ToString().Length != 4) {
Console.WriteLine("Error: the pincode needs to be 4 digits, try it again");
}
else if (firstPincode.Equals(secondPincode)) {
return firstPincode;
}
else {
Console.WriteLine("Error: the two entered pincodes didn't match, try it again");
}
}
}
/// <summary>
/// The deposit menu
/// </summary>
private void Deposit() {
Console.WriteLine("Deposit \n How much do you like to deposit?");
var givenAmmount = InputHandling.CheckNumber();
queryAccess.accountTable.ChangeBalance((a) => a.AccountId == LoggedInUser.AccountId, givenAmmount, (a) => a.Money += givenAmmount);
Console.WriteLine("The money has been deposit");
}
/// <summary>
/// The change pincode menu
/// </summary>
private void ChangePincode() {
Console.WriteLine("Change Pincode");
var choosenPincode = this.PincodeConfirmation("What would you like to change your pincode to?");
queryAccess.accountTable.ChangePincode(LoggedInUser.AccountId, choosenPincode);
Console.WriteLine("Pincode has been changed");
}
/// <summary>
/// The Withdraw menu
/// </summary>
private void Withdraw() {
Console.WriteLine("Withdraw \n How much do you like to draw?");
var givenAmmount = InputHandling.CheckNumber();
if(this.IsGivenAmmountHigherThanBalance(givenAmmount)) {
queryAccess.accountTable.ChangeBalance((a) => a.AccountId == LoggedInUser.AccountId, givenAmmount, (a) => a.Money -= givenAmmount);
Console.WriteLine("Money has been Withdrawn");
}
else {
Console.WriteLine("Error: given ammount should be higher than the current balance");
this.BackRetryExit(() => this.MainMenuOptions(), () => this.Withdraw());
}
}
/// <summary>
/// The transfer menu
/// </summary>
private void Transfer() {
Console.WriteLine("Transfer \n To which account to transfer money?");
var givenBankNumber = InputHandling.CheckNumber();
if (this.queryAccess.accountTable.CheckIfBankNumberExists(givenBankNumber)) {
Console.WriteLine("How much to deposit?");
var givenAmmount = InputHandling.CheckNumber();
if (this.IsGivenAmmountHigherThanBalance(givenAmmount)) {
this.queryAccess.accountTable.ChangeBalance((a) => a.BankNumber == givenBankNumber, givenAmmount, (a) => a.Money += givenAmmount);
this.queryAccess.accountTable.ChangeBalance((a) => a.AccountId == LoggedInUser.AccountId, givenAmmount, (a) => a.Money -= givenAmmount);
Console.WriteLine("Money has been transfered");
return;
}
else {
Console.WriteLine("Error: given ammount is lower than balance, current balance: {0}", LoggedInUser.Balance);
this.BackRetryExit(() => this.MainMenuOptions(), () => this.Transfer());
return;
}
}
Console.WriteLine("Error: Bank number does not exist");
this.BackRetryExit(() => this.MainMenuOptions(), () => this.Transfer());
}
/// <summary>
/// Checks if the given ammount is higher than user's current balance
/// </summary>
/// <param name="ammount">ammount of money that has been typed by the user</param>
/// <returns></returns>
private bool IsGivenAmmountHigherThanBalance(int ammount) {
if(ammount <= LoggedInUser.Balance && ammount > 0) {
return true;
}
return false;
}
/// <summary>
/// The menu for check balance
/// </summary>
private void SeeBalance() {
Console.WriteLine("The current balance is: {0}", LoggedInUser.Balance);
}
}
}
<file_sep>/The-ATM-Machine/LoggedInUser/LoggedInUser.cs
using The_ATM_Machine.Queries;
namespace The_ATM_Machine {
/// <summary>
/// Information about the logged in user
/// </summary>
public class LoggedInUser {
private static int accountId; //id of the account Table
private static string name;
private static bool isUserLoggedIn = false;
public static int AccountId { get => accountId; set => accountId = value; }
public static string Name { get => name; set => name = value; }
public static int Balance { get => new QueryAccess().accountTable.GetAccountInformation(accountId, (a) => a.Money); }
public static int Pincode { get => new QueryAccess().accountTable.GetAccountInformation(accountId, (a) => a.Pincode);}
private LoggedInUser() {
}
public static void LogInUser(string name, int accountId) {
if (!isUserLoggedIn) {
LoggedInUser.name = name;
LoggedInUser.accountId = accountId;
LoggedInUser.isUserLoggedIn = true;
}
}
}
}
<file_sep>/The-ATM-Machine/Queries/AccountQueries.cs
using System;
using System.Linq;
namespace The_ATM_Machine.Queries {
/// <summary>
/// Handles queries related to the account table
/// </summary>
public class AccountQueries {
TheDatabase database = new TheDatabase();
public bool CheckIfBankNumberExists(int bankNumber) {
var res = database.database.Accounts.Where(a => a.BankNumber == bankNumber).FirstOrDefault();
if(res == null) {
return false;
}
return true;
}
public void AddAccount(Account account) {
database.database.Accounts.Add(account);
database.database.SaveChanges();
}
public int GetAccountId(int bankNumber) {
var res = database.database.Accounts.Where(a => a.BankNumber == bankNumber).FirstOrDefault();
return res.AccountId;
}
public bool IsPincodeCorrect(int bankNumber, int pincode) {
var res = database.database.Accounts.Where(a => a.BankNumber == bankNumber).FirstOrDefault();
if(res.Pincode == pincode) {
return true;
}
return false;
}
public void ChangeBalance(Func<Account,bool> wherePredicate, int ammount, Action<Account> predicate) {
var account = database.database.Accounts.Where(wherePredicate).FirstOrDefault();
predicate(account);
database.database.SaveChanges();
}
public void ChangePincode(int accountId, int pincode) {
database.database.Accounts.Where(a => a.AccountId == accountId).FirstOrDefault().Pincode = pincode;
database.database.SaveChanges();
}
public int GetAccountInformation(int accountId, Func<Account, dynamic> predicate) {
var res = database.database.Accounts.Where((a) => a.AccountId == accountId).FirstOrDefault();
return predicate(res);
}
}
}
<file_sep>/The-ATM-Machine/Queries/QueryAccess.cs
namespace The_ATM_Machine.Queries {
/// <summary>
/// For access to both tables (the whole database)
/// </summary>
public class QueryAccess {
public AccountQueries accountTable = new AccountQueries();
public UserQueries user = new UserQueries();
}
}
<file_sep>/The-ATM-Machine/Queries/Database.cs
namespace The_ATM_Machine {
public class TheDatabase {
public DatabaseContext database;
public TheDatabase() {
database = new DatabaseContext();
}
}
}<file_sep>/The-ATM-Machine/Queries/Models.cs
using Microsoft.EntityFrameworkCore;
using System.ComponentModel.DataAnnotations;
namespace The_ATM_Machine {
public class DatabaseContext : DbContext {
public DbSet<User> Users { get; set; }
public DbSet<Account> Accounts { get; set; }
protected override void OnConfiguring(DbContextOptionsBuilder op) {
op.UseSqlite("Data Source=atmDB.db");
}
}
public class User {
public int UserId { get; set; }
public string Name { get; set; }
public string Surname { get; set; }
public int AccountId { get; set; }
public Account Account { get; set; }
}
public class Account {
public int AccountId { get; set; }
public int BankNumber { get; set; }
[MaxLength(4)]
public int Pincode { get; set; }
public int Money { get; set; }
public User User { get; set; }
}
}<file_sep>/README.md
# Personal project
ATM (Automated teller machine)
# Features
- Login system
- Withdraw money and deposit money
- Check current balance
- Change pincode
- Transfer money to another bank account

# How to start
Run the client file.
# Type
- Console application
# Tools
- Entity Framework
- LINQ (Enumerable)
- SQLite database
<file_sep>/The-ATM-Machine/Queries/UserQueries.cs
using System;
using System.Linq;
namespace The_ATM_Machine.Queries {
/// <summary>
/// Queries related to the user table
/// </summary>
public class UserQueries {
TheDatabase database = new TheDatabase();
public void AddUser(User user) {
this.database.database.Users.Add(user);
this.database.database.SaveChanges();
}
public Tuple<string,int,int> GetUserInformation(int bankNumber) {
var res = database.database.Users.Join(database.database.Accounts, (u) => u.AccountId, (a) => a.AccountId, (ures, aRes) =>
new Tuple<string,int,int>(ures.Name, aRes.AccountId, aRes.BankNumber))
.Where((tables) => tables.Item3 == bankNumber).FirstOrDefault();
return res;
}
}
}
<file_sep>/The-ATM-Machine/Machine/InputHandling.cs
using System;
namespace The_ATM_Machine {
/// <summary>
/// Verifies if the number that has been typed by the user, is correct
/// </summary>
public class InputHandling {
public static int CheckNumber() {
var givenNumber = 0;
while (true) {
var answer = Int32.TryParse(Console.ReadLine(), out givenNumber);
if (answer && givenNumber > 0){
return givenNumber;
}
Console.WriteLine("Error: enter a valid number above the 0");
}
}
}
}
<file_sep>/The-ATM-Machine/Client.cs
namespace The_ATM_Machine {
/// <summary>
/// Start of the program
/// </summary>
public class Client {
static void Main(string[] args) {
var atm = new Machine();
atm.StartUpMenuOptions(); //when the user is not logged in
atm.MainMenuOptions(); //when the user is logged in
}
}
}
| e4ed3c9aa895ffea20ebcf7abfad2f0411b06f2a | [
"Markdown",
"C#"
] | 11 | C# | brandedux/atm-sim | dca0c14ce8fb721ecae4b85e3ced3919a1cc4dc4 | 79c938408d986b70bd611bad7f406dfd91c3b567 | |
refs/heads/master | <repo_name>isabella232/canoe<file_sep>/tests/unit/test_handler.py
# coding: utf-8
import io
import os
import sys
import pytest
from unittest.mock import Mock
import xml.etree.ElementTree as ElementTree
CWD = os.path.dirname(os.path.realpath(__file__)) + "/../../"
sys.path.insert(0, os.path.join(CWD, ''))
from canoe import app # noqa
@pytest.fixture()
def kayako():
client = Mock()
departments = """<?xml version="1.0" encoding="UTF-8"?>
<departments>
<department>
<id><![CDATA[1]]></id>
<title><![CDATA[Project Name]]></title>
</department>
<department>
<id><![CDATA[2]]></id>
<title><![CDATA[Customer 2]]></title>
<parentdepartmentid><![CDATA[1]]></parentdepartmentid>
</department>
<department>
<id><![CDATA[3]]></id>
<title><![CDATA[Customer 3]]></title>
<parentdepartmentid><![CDATA[1]]></parentdepartmentid>
</department>
<department>
<id><![CDATA[4]]></id>
<title><![CDATA[Customer 4]]></title>
<parentdepartmentid><![CDATA[1]]></parentdepartmentid>
</department>
<department>
<id><![CDATA[5]]></id>
<title><![CDATA[Other Main Department]]></title>
</department>
</departments>
"""
client.list_departments.return_value = ElementTree.fromstring(departments)
tickets = """<?xml version="1.0" encoding="UTF-8"?>
<tickets>
<ticket id="273" flagtype="5">
<displayid><![CDATA[MAB-597-12345]]></displayid>
</ticket>
<ticket id="274" flagtype="5">
<displayid><![CDATA[JAB-293-54321]]></displayid>
</ticket>
</tickets>
"""
client.list_open_tickets.return_value = ElementTree.fromstring(tickets)
posts = """<?xml version="1.0" encoding="UTF-8"?>
<tickets>
<ticket id="277" flagtype="5">
<displayid><![CDATA[CYA-293-12345]]></displayid>
<departmentid><![CDATA[20]]></departmentid>
<userorganization><![CDATA[Customer Name]]></userorganization>
<ownerstaffname><![CDATA[Staff Owner]]></ownerstaffname>
<fullname><![CDATA[Customer]]></fullname>
<email><![CDATA[<EMAIL>]]></email>
<subject><![CDATA[Mayday Mayday]]></subject>
<posts>
<post>
<id><![CDATA[3]]></id>
<ticketpostid><![CDATA[1496]]></ticketpostid>
<ticketid><![CDATA[277]]></ticketid>
<dateline><![CDATA[1552419863]]></dateline>
<fullname><![CDATA[Sender FullName (customer)]]></fullname>
<email><![CDATA[<EMAIL>]]></email>
<contents><![CDATA[Thanks mate
]]></contents>
</post>
<post>
<id><![CDATA[2]]></id>
<ticketpostid><![CDATA[1495]]></ticketpostid>
<ticketid><![CDATA[277]]></ticketid>
<dateline><![CDATA[1552418863]]></dateline>
<fullname><![CDATA[Sender FullName]]></fullname>
<email><![CDATA[<EMAIL>]]></email>
<contents><![CDATA[Any time!
Thank you,
Your support
]]></contents>
</post>
<post>
<id><![CDATA[1]]></id>
<ticketpostid><![CDATA[1488]]></ticketpostid>
<ticketid><![CDATA[277]]></ticketid>
<dateline><![CDATA[1552317114]]></dateline>
<fullname><![CDATA[Sender FullName (customer)]]></fullname>
<email><![CDATA[<EMAIL>]]></email>
<contents><![CDATA[This fixed it,
Thank you!
]]></contents>
</post>
</posts>
</ticket>
</tickets>
"""
client.get_ticket.return_value = ElementTree.fromstring(posts)
return client
@pytest.fixture()
def slack(monkeypatch):
client = Mock()
monkeypatch.setattr('canoe.app.slack_client', client)
return client
@pytest.fixture()
def seed_event():
return {'type': 'seed'}
@pytest.fixture()
def context():
return {}
def test_list_relevant_department_ids(kayako):
ids = app.list_relevant_department_ids(kayako, 'Project Name')
assert ['1', '2', '3', '4'] == list(ids)
def test_seed_handler(seed_event, context, kayako, monkeypatch):
session = Mock()
monkeypatch.setattr('canoe.app.session', session)
sqs = session.resource.return_value
queue = sqs.Queue.return_value
monkeypatch.setattr('canoe.app.kayako', kayako)
monkeypatch.setenv('CANOE_ROOT_PROJECT_NAME', 'Project Name')
app.seed_handler(seed_event, context)
queue.send_messages.assert_called_with(
Entries=[
{'Id': '1', 'MessageBody': '{"department_id": "1"}'},
{'Id': '2', 'MessageBody': '{"department_id": "2"}'},
{'Id': '3', 'MessageBody': '{"department_id": "3"}'},
{'Id': '4', 'MessageBody': '{"department_id": "4"}'}
]
)
@pytest.fixture()
def sqs_departments_event():
return {
'Records': [
{
'body': '{"department_id": "2"}',
}
]
}
def test_distribute_departments_tickets_handler(
sqs_departments_event, context, kayako, monkeypatch):
session = Mock()
monkeypatch.setattr('canoe.app.session', session)
sqs = session.resource.return_value
queue = sqs.Queue.return_value
monkeypatch.setattr('canoe.app.kayako', kayako)
app.distribute_departments_tickets_handler(sqs_departments_event, context)
queue.send_messages.assert_called_with(
Entries=[
{'Id': '273', 'MessageBody': '{"ticket_id": "273"}'},
{'Id': '274', 'MessageBody': '{"ticket_id": "274"}'}
]
)
@pytest.fixture()
def sqs_check_tickets_event():
return {
'Records': [
{
'body': '{"ticket_id": "273"}',
}
]
}
def test_check_ticket_handler(
sqs_check_tickets_event, context, kayako, monkeypatch):
s3 = Mock()
monkeypatch.setattr('canoe.app.s3', s3)
state = """<?xml version="1.0" encoding="UTF-8"?>
<tickets>
<ticket id="277" flagtype="5">
<displayid><![CDATA[CYA-293-12345]]></displayid>
<departmentid><![CDATA[20]]></departmentid>
<userorganization><![CDATA[Customer Name]]></userorganization>
<ownerstaffname><![CDATA[Staff Owner]]></ownerstaffname>
<fullname><![CDATA[Customer]]></fullname>
<email><![CDATA[<EMAIL>]]></email>
<subject><![CDATA[Mayday Mayday]]></subject>
<posts>
<post>
<id><![CDATA[2]]></id>
<ticketpostid><![CDATA[1495]]></ticketpostid>
<ticketid><![CDATA[277]]></ticketid>
<dateline><![CDATA[1552418863]]></dateline>
<fullname><![CDATA[Sender FullName]]></fullname>
<email><![CDATA[<EMAIL>]]></email>
<contents><![CDATA[Any time!
Thank you,
Your support
]]></contents>
</post>
<post>
<id><![CDATA[1]]></id>
<ticketpostid><![CDATA[1488]]></ticketpostid>
<ticketid><![CDATA[277]]></ticketid>
<dateline><![CDATA[1552317114]]></dateline>
<fullname><![CDATA[Sender FullName (customer)]]></fullname>
<email><![CDATA[<EMAIL>]]></email>
<contents><![CDATA[This fixed it,
Thank you!
]]></contents>
</post>
</posts>
</ticket>
</tickets>
"""
s3_obj = {'Body': io.StringIO(state)}
s3.get_object.return_value = s3_obj
session = Mock()
monkeypatch.setattr('canoe.app.session', session)
sqs = session.resource.return_value
queue = sqs.Queue.return_value
monkeypatch.setattr('canoe.app.kayako', kayako)
app.check_ticket_handler(sqs_check_tickets_event, context)
queue.send_messages.assert_called_with(
Entries=[
{
'Id': '0',
'MessageBody': '{"type": "new_post", "object": {"dateline": "1552419863", "fullname": "Sender FullName (customer)", "email": "<EMAIL>", "contents": "Thanks mate\\n\\n ", "displayid": "CYA-293-12345", "userorganization": "Customer Name", "subject": "Mayday Mayday", "ticket_id": "273"}}' # noqa: E501
}
]
)
def test_diff_new_posts_empty_state():
posts = """<?xml version="1.0" encoding="UTF-8"?>
<tickets>
<ticket>
<posts>
<post>
<ticketpostid><![CDATA[1496]]></ticketpostid>
<dateline><![CDATA[1552419863]]></dateline>
</post>
<post>
<ticketpostid><![CDATA[1495]]></ticketpostid>
<dateline><![CDATA[1552418863]]></dateline>
</post>
<post>
<ticketpostid><![CDATA[1488]]></ticketpostid>
<dateline><![CDATA[1552317114]]></dateline>
</post>
</posts>
</ticket>
</tickets>
"""
diff = app.diff_new_posts(ElementTree.fromstring(posts), None)
diff_xml = [ElementTree.tostring(el, encoding="unicode") for el in diff]
assert diff_xml == [
'<post>\n'
' <ticketpostid>1488</ticketpostid>\n'
' <dateline>1552317114</dateline>\n'
' </post>\n'
' ',
'<post>\n'
' <ticketpostid>1495</ticketpostid>\n'
' <dateline>1552418863</dateline>\n'
' </post>\n'
' ',
'<post>\n'
' <ticketpostid>1496</ticketpostid>\n'
' <dateline>1552419863</dateline>\n'
' </post>\n'
' '
]
def test_diff_new_posts_empty_many_items():
posts = """<?xml version="1.0" encoding="UTF-8"?>
<tickets>
<ticket>
<posts>
<post>
<ticketpostid><![CDATA[1496]]></ticketpostid>
<dateline><![CDATA[1552419863]]></dateline>
</post>
<post>
<ticketpostid><![CDATA[1495]]></ticketpostid>
<dateline><![CDATA[1552418863]]></dateline>
</post>
<post>
<ticketpostid><![CDATA[1488]]></ticketpostid>
<dateline><![CDATA[1552317114]]></dateline>
</post>
</posts>
</ticket>
</tickets>
"""
state = """<?xml version="1.0" encoding="UTF-8"?>
<tickets>
<ticket>
<posts>
<post>
<ticketpostid><![CDATA[1488]]></ticketpostid>
<dateline><![CDATA[1552317114]]></dateline>
</post>
</posts>
</ticket>
</tickets>
"""
diff = app.diff_new_posts(ElementTree.fromstring(posts), ElementTree.fromstring(state))
diff_xml = [ElementTree.tostring(el, encoding="unicode") for el in diff]
assert diff_xml == [
'<post>\n'
' <ticketpostid>1495</ticketpostid>\n'
' <dateline>1552418863</dateline>\n'
' </post>\n'
' ',
'<post>\n'
' <ticketpostid>1496</ticketpostid>\n'
' <dateline>1552419863</dateline>\n'
' </post>\n'
' '
]
@pytest.fixture()
def updates_event():
return {
'Records': [
{
'body': '{"type": "new_post", "object": {"dateline": "1552419863", "fullname": "Sender FullName (customer)", "email": "<EMAIL>", "contents": "Thanks mate\\n\\n ", "displayid": "CYA-293-12345", "userorganization": "Customer Name", "subject": "Mayday Mayday", "ticket_id": "273"}}' # noqa: E501
}
]
}
def test_updates_notifications_handler(monkeypatch, slack, updates_event, context):
app.updates_notifications_handler(updates_event, context)
slack.chat_postMessage.assert_called_with(
channel='PROJECTID',
text='[CYA-293-12345]: Mayday Mayday\n@here Sender FullName (customer) left a comment on a ticket',
blocks=[
{
'type': 'section',
'text': {
'type': 'mrkdwn',
'text': '<https://kayako-srv.com?/Tickets/Ticket/View/273|[CYA-293-12345]: Mayday Mayday>'
}
},
{
'type': 'section',
'text': {
'type': 'mrkdwn',
'text': '@here Sender FullName (customer) left a comment on a ticket'
}
}
]
)
<file_sep>/canoe/app.py
import boto3
import json
import os
import logging
import sys
import bisect
import itertools
import slack
from xml.etree import ElementTree
CWD = os.path.dirname(os.path.realpath(__file__))
sys.path.insert(0, os.path.join(CWD, 'lib'))
from kayako import Kayako # noqa: E402
LOG_LEVEL = logging.INFO
boto3.set_stream_logger('', LOG_LEVEL)
logger = logging.getLogger()
logger.setLevel(LOG_LEVEL)
# Global variables are reused across execution contexts (if available)
session = boto3.Session()
s3 = session.client('s3')
kayako = Kayako(os.getenv('CANOE_KAYAKO_API_URL'),
os.getenv('CANOE_KAYAKO_API_KEY'),
os.getenv('CANOE_KAYAKO_SECRET_KEY'))
SLACK_CHANNEL_ID = os.getenv('CANOE_SLACK_CHANNEL_ID')
slack_client = slack.WebClient(token=os.getenv('CANOE_SLACK_API_TOKEN'))
def seed_handler(event, context):
if event.get('type', None) != 'seed':
logger.warning(f'unexpected event: {event}')
return
project_name = os.getenv('CANOE_ROOT_PROJECT_NAME')
dep_ids = list_relevant_department_ids(kayako, project_name)
queue_url = os.getenv('CANOE_CHECK_DEPARTMENT_QUEUE_URL')
sqs = session.resource('sqs')
queue = sqs.Queue(queue_url)
messages = sqs_messages(dep_ids)
if not messages:
logger.warning('no messages to send')
return
send_messages(queue, messages)
def send_messages(queue, items, batch_size=10):
iter_items = [iter(items)] * batch_size
for batch in itertools.zip_longest(*iter_items, fillvalue=None):
batch = list(filter(None, batch))
queue.send_messages(Entries=batch)
def distribute_departments_tickets_handler(event, context):
ticket_ids = []
for record in event['Records']:
message = json.loads(record['body'])
department_id = message['department_id']
tickets = kayako.list_open_tickets(department_id)
for ticket in tickets.findall('.//ticket'):
ticket_id = ticket.get('id')
ticket_ids.append(ticket_id)
queue_url = os.getenv('CANOE_CHECK_TICKET_QUEUE_URL')
sqs = session.resource('sqs')
queue = sqs.Queue(queue_url)
messages = check_ticket_messages(ticket_ids)
send_messages(queue, messages)
# we are including the top level department and sub departments as well
def list_relevant_department_ids(kayako, project_name):
departments = kayako.list_departments()
parent_el_ids = departments.findall(f".//department/title[.='{project_name}']../id")
for parent_el_id in parent_el_ids:
yield parent_el_id.text
xpath = f".//department/parentdepartmentid[.='{parent_el_id.text}']../id"
for dep_id_el in departments.findall(xpath):
yield dep_id_el.text
def check_ticket_handler(event, context):
tickets_updates = []
for record in event['Records']:
message = json.loads(record['body'])
ticket_id = message['ticket_id']
state = get_ticket_state(ticket_id)
ticket = kayako.get_ticket(ticket_id)
new_posts = diff_new_posts(ticket, state)
updates = list(ticket_updates(ticket_id, ticket, new_posts))
tickets_updates.extend(updates)
if updates:
save_ticket_state(ticket_id, ticket)
if not is_in_learning_mode():
queue_url = os.getenv('CANOE_TICKETS_UPDATES_QUEUE_URL')
sqs = session.resource('sqs')
queue = sqs.Queue(queue_url)
messages = tickets_updates_messages(tickets_updates)
send_messages(queue, messages)
def is_in_learning_mode():
return os.getenv('CANOE_LEARNING_MODE') == 'true'
def updates_notifications_handler(event, context):
for record in event['Records']:
body = json.loads(record['body'])
if body['type'] == 'new_post':
new_post = body['object']
text = message_text(new_post)
blocks = message_blocks(new_post)
slack_client.chat_postMessage(
channel=SLACK_CHANNEL_ID,
text=text,
blocks=blocks)
def message_text(new_post):
tmpl = ('[{displayid}]: {subject}\n'
'@here {fullname} left a comment on a ticket')
return tmpl.format(**new_post)
def message_blocks(new_post):
portal_uri = os.getenv('CANOE_KAYAKO_UI_URL')
ticket_link = '{portal_uri}?/Tickets/Ticket/View/{ticket_id}'.format(
portal_uri=portal_uri, **new_post)
return [
{
'type': 'section',
'text': {
'type': 'mrkdwn',
'text': '<{link}|[{displayid}]: {subject}>'.format(link=ticket_link, **new_post)
}
},
{
'type': 'section',
'text': {
'type': 'mrkdwn',
'text': '@here {fullname} left a comment on a ticket'.format(**new_post)
}
}
]
def save_ticket_state(ticket_id, ticket):
bucket = tickets_state_bucket()
key = ticket_state_key(ticket_id)
xml = ElementTree.tostring(ticket, encoding="utf-8")
s3.put_object(Bucket=bucket, Key=key, Body=xml)
def diff_new_posts(ticket, state):
state_dateline = latest_post_dateline(state)
posts = ticket.findall('.//posts/post')
datelines = [int(post.find('dateline').text) for post in posts]
datelined_posts = list(zip(datelines, posts))
datelined_posts.sort(key=lambda p: p[0])
datelines, posts = zip(*datelined_posts)
pos = bisect.bisect_right(datelines, state_dateline)
return posts[pos:]
def ticket_updates(ticket_id, ticket, posts):
fields = ['displayid', 'userorganization', 'subject']
update_base = extract_fields(ticket, fields, './/ticket/{}')
update_base['ticket_id'] = ticket_id
for post in posts:
ticket_fields = ['dateline', 'fullname', 'email', 'contents']
ticket_update = extract_fields(post, ticket_fields)
ticket_update.update(update_base)
yield ticket_update
def extract_fields(element, fields, path_tmpl=None):
def format_path(p):
return path_tmpl.format(p) if path_tmpl else p
return {field: element.find(format_path(field)).text for field in fields}
def latest_post_dateline(state):
if not state:
return 0
dateline_els = state.findall('.//post/dateline')
return max([int(dl.text) for dl in dateline_els])
def get_ticket_state(ticket_id):
state = read_ticket_state(ticket_id)
if state:
return ElementTree.parse(state)
def read_ticket_state(ticket_id):
key = ticket_state_key(ticket_id)
bucket = tickets_state_bucket()
try:
s3_object = s3.get_object(Bucket=bucket, Key=key)
return s3_object['Body']
except s3.exceptions.NoSuchKey:
logger.info(f'No state found {bucket}/{key}')
def ticket_state_key(ticket_id):
return f'tickets/{ticket_id}.xml'
def tickets_state_bucket():
return os.getenv('CANOE_TICKETS_STATE_BUCKET')
def sqs_messages(department_ids):
return [
{
'Id': dep_id,
'MessageBody': json.dumps({'department_id': dep_id})
}
for dep_id in department_ids
]
def check_ticket_messages(ticket_ids):
return [
{
'Id': ticket_id,
'MessageBody': json.dumps({'ticket_id': ticket_id})
}
for ticket_id in ticket_ids
]
def tickets_updates_messages(updates):
return [
{
'Id': str(index),
'MessageBody': json.dumps({
'type': 'new_post',
'object': update
})
}
for index, update in enumerate(updates)
]
<file_sep>/canoe/lib/kayako.py
import base64
import hmac
import random
import requests
from xml.etree import ElementTree
from requests.auth import AuthBase
from urllib.parse import urlsplit, parse_qsl, urlencode
class KayakoAuth(AuthBase):
def __init__(self, api_key, secret_key):
self._api_key = api_key
self._secret_key = secret_key.encode('utf-8')
def __call__(self, request):
salt = str(random.getrandbits(32)).encode('utf-8')
digest = hmac.digest(self._secret_key, msg=salt, digest='sha256')
signature = base64.encodebytes(digest).replace(b'\n', b'')
url_split = urlsplit(request.url)
query = dict(parse_qsl(url_split.query))
query['apikey'] = self._api_key
query['salt'] = salt
query['signature'] = signature
auth_url_split = url_split._replace(query=urlencode(query))
request.url = auth_url_split.geturl()
return request
class Kayako:
def __init__(self, url, api_key, secret_key):
self._url = url
self._session = requests.Session()
self._session.auth = KayakoAuth(api_key, secret_key)
def list_departments(self):
text = self.request('get', '/Base/Department')
return ElementTree.fromstring(text)
def list_open_tickets(self, department_id):
action = f'/Tickets/Ticket/ListAll/{department_id}/1/-1/-1/-1/-1/ticketid/ASC'
text = self.request('get', action)
return ElementTree.fromstring(text)
def get_ticket(self, ticket_id):
action = f'/Tickets/Ticket/{ticket_id}'
text = self.request('get', action)
return ElementTree.fromstring(text)
def request(self, method, action, params=None, **kwargs):
params = params or {}
params['e'] = action
response = self._session.request(
method, self._url, params=params, **kwargs)
response.raise_for_status()
return response.text
<file_sep>/build.sh
STACK_NAME=canoe-helpdesk
S3_BUCKET=my-bucket #obtain it from internal docs
gmake build SERVICE=canoe
aws --region eu-west-2 cloudformation package \
--template-file template.yaml \
--output-template-file packaged.yaml \
--s3-bucket $S3_BUCKET
aws --region eu-west-2 cloudformation deploy \
--template-file $PWD/packaged.yaml \
--stack-name $STACK_NAME \
--parameter-overrides "file://$PWD/env.json" \
--capabilities CAPABILITY_IAM
| 9a3d954c59db497c320de779404c2cdbc9ff4f05 | [
"Python",
"Shell"
] | 4 | Python | isabella232/canoe | de16e084335c72501dadaf79e425e1b9b75099bb | 84e2634727e1138bf1efb10b2f3c1b5e685eb7c7 | |
refs/heads/master | <repo_name>MehdiNezamipour/TapsellTaskWithKotlin<file_sep>/AdMediator/src/main/java/com/nezamipour/mehdi/admediator/mainclasses/AdMediator.kt
package com.nezamipour.mehdi.admediator.mainclasses
import android.app.Activity
import android.app.Application
import android.content.Context
import android.util.Log
import com.chartboost.sdk.Chartboost
import com.nezamipour.mehdi.admediator.mainclasses.companies.ChartBoostManager
import com.nezamipour.mehdi.admediator.mainclasses.companies.CompanyManager
import com.nezamipour.mehdi.admediator.mainclasses.companies.TapSellManager
import com.nezamipour.mehdi.admediator.mainclasses.companies.UnityAdsManager
import com.nezamipour.mehdi.admediator.mainclasses.listeners.AdRequestCallback
import com.nezamipour.mehdi.admediator.mainclasses.listeners.AdShowCallback
import com.nezamipour.mehdi.admediator.model.AdConfig
import com.nezamipour.mehdi.admediator.model.AppConfig
import com.nezamipour.mehdi.admediator.network.AdMediatorService
import com.nezamipour.mehdi.admediator.utils.Constants
import com.unity3d.ads.UnityAds
import kotlinx.coroutines.*
import kotlinx.coroutines.Dispatchers.IO
class AdMediator {
companion object {
private val allCompanies: HashMap<String, CompanyManager> = hashMapOf()
private val TAG = "AdMediator"
private lateinit var appConfig: AppConfig
private lateinit var adConfig: AdConfig
internal lateinit var zoneType: String
internal var adFound: Boolean = false
internal var sAdId: String? = null
fun initialize(application: Application, adMediatorAppId: String) {
getAppConfig(adMediatorAppId)
// initialization adNetworks base on appConfig
if (appConfig.adNetworks.tapsell != null) {
TapSellManager.initial(application, appConfig.adNetworks.tapsell!!)
allCompanies["Tapsell"] = TapSellManager
}
if (appConfig.adNetworks.unityAds != null) {
UnityAds.initialize(application, appConfig.adNetworks.unityAds)
allCompanies["UnityAds"] = UnityAdsManager
}
if (appConfig.adNetworks.chartboost != null) {
Chartboost.startWithAppId(application, appConfig.adNetworks.chartboost, null)
allCompanies["Chartboost"] = ChartBoostManager
}
}
private fun getAppConfig(appId: String) {
try {
CoroutineScope(IO).launch {
appConfig = AdMediatorService.getAppConfig(appId)
}
} catch (e: Exception) {
e.printStackTrace()
Log.d(TAG, "getAppConfig: " + e.message)
}
Log.d(TAG, "getAppConfig : " + appConfig.adNetworks.tapsell)
Log.d(TAG, "getAppConfig : " + adConfig.waterfall.toString())
}
private fun getAdConfigBaseOnZoneId(zoneId: String) {
try {
CoroutineScope(IO).launch {
adConfig = AdMediatorService.getAdConfig(zoneId)
zoneType = adConfig.zoneType
}
} catch (e: Exception) {
e.printStackTrace()
Log.d(TAG, "getAdConfigBaseOnZoneId : " + e.message)
}
}
fun requestAd(
context: Context,
adMediatorZoneId: String,
adRequestCallback: AdRequestCallback
) {
// TODO : get ad config with new waterfall if not exist waterfall with this zoneId in catch
getAdConfigBaseOnZoneId(zoneId = adMediatorZoneId)
loadAd(context, adRequestCallback)
}
private fun loadAd(
context: Context,
adRequestCallback: AdRequestCallback
) {
val parentJob = CoroutineScope(IO).launch {
withTimeoutOrNull(adConfig.waterfall[0].timeout) {
if (!adFound)
try {
//delay(2100)
Log.d(TAG, "loadAd: ${adConfig.waterfall[0].zoneId}")
allCompanies[adConfig.waterfall[0].adNetwork]?.requestAd(
context,
adConfig.waterfall[0].zoneId,
adRequestCallback
)
} catch (e: Exception) {
Log.d(TAG, "loadAd: exception message = ${e.message}")
}
else
cancel()
}
withTimeoutOrNull(adConfig.waterfall[1].timeout) {
if (!adFound)
try {
Log.d(TAG, "loadAd: ${adConfig.waterfall[1].zoneId}")
allCompanies[adConfig.waterfall[1].adNetwork]?.requestAd(
context,
adConfig.waterfall[1].zoneId,
adRequestCallback
)
} catch (e: Exception) {
Log.d(TAG, "loadAd: exception message = ${e.message}")
}
else
cancel()
}
withTimeoutOrNull(adConfig.waterfall[2].timeout) {
if (!adFound)
try {
Log.d(TAG, "loadAd: ${adConfig.waterfall[2].zoneId}")
allCompanies[adConfig.waterfall[2].adNetwork]?.requestAd(
context,
adConfig.waterfall[2].zoneId,
adRequestCallback
)
} catch (e: Exception) {
Log.d(TAG, "loadAd: exception message = ${e.message}")
}
else
cancel()
}
}
}
fun showAd(
activity: Activity,
zoneId: String,
adId: String,
adShowCallback: AdShowCallback
) {
when (zoneId) {
Constants.TAPSELL_INTERSTITIAL_BANNER, Constants.TAPSELL_INTERSTITIAL_VIDEO, Constants.TAPSELL_REWARDED_VIDEO ->
sAdId?.let {
allCompanies["Tapsell"]?.showAd(
activity,
zoneId,
it,
adShowCallback
)
}
Constants.CHART_BOOST_INTERSTITIAL_BANNER, Constants.CHART_BOOST_INTERSTITIAL_VIDEO ->
sAdId?.let {
allCompanies["Chartboost"]?.showAd(
activity,
zoneId,
it,
adShowCallback
)
}
Constants.CHART_BOOST_REWARDED_VIDEO -> sAdId?.let {
allCompanies["Chartboost"]?.showAd(
activity,
zoneId,
it,
adShowCallback
)
}
Constants.UNITY_AD_INTERSTITIAL_BANNER, Constants.UNITY_AD_INTERSTITIAL_VIDEO, Constants.UNITY_AD_REWARDED_VIDEO ->
sAdId?.let {
allCompanies["UnityAds"]?.showAd(
activity,
zoneId,
it,
adShowCallback
)
}
else -> adShowCallback.onError("showAd : network not exist")
}
}
}
}<file_sep>/app/src/main/java/com/nezamipour/mehdi/tapselltaskwithkotlin/InterstitialBannerActivity.kt
package com.nezamipour.mehdi.tapselltaskwithkotlin
import android.content.Context
import android.content.Intent
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.Toast
import com.nezamipour.mehdi.admediator.mainclasses.AdMediator
import com.nezamipour.mehdi.admediator.mainclasses.listeners.AdRequestCallback
import com.nezamipour.mehdi.admediator.mainclasses.listeners.AdShowCallback
import com.nezamipour.mehdi.admediator.utils.Constants
import com.nezamipour.mehdi.tapselltaskwithkotlin.databinding.ActivityInterstitialBannerBinding
import kotlinx.coroutines.CoroutineScope
import kotlinx.coroutines.Dispatchers.IO
import kotlinx.coroutines.launch
class InterstitialBannerActivity : AppCompatActivity() {
private lateinit var binding: ActivityInterstitialBannerBinding
private lateinit var adId: String
companion object {
fun newIntent(context: Context): Intent {
return Intent(context, InterstitialBannerActivity::class.java)
}
}
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = ActivityInterstitialBannerBinding.inflate(layoutInflater)
setContentView(binding.root)
binding.buttonRequestAd.setOnClickListener {
CoroutineScope(IO).launch {
AdMediator.requestAd(
context = this@InterstitialBannerActivity,
Constants.AD_MEDIATOR_INTERSTITIAL_BANNER,
object : AdRequestCallback {
override fun onSuccess(adId: String?) {
binding.buttonShowAd.isEnabled = true
if (adId != null) {
[email protected] = adId
}
}
override fun error(message: String?) {
Toast.makeText(
this@InterstitialBannerActivity,
message,
Toast.LENGTH_SHORT
)
.show()
}
})
}
}
binding.buttonShowAd.setOnClickListener {
AdMediator.showAd(
this,
Constants.AD_MEDIATOR_INTERSTITIAL_BANNER,
adId,
object : AdShowCallback {
override fun onOpened() {
TODO("Not yet implemented")
}
override fun onClosed() {
TODO("Not yet implemented")
}
override fun onRewarded() {
TODO("Not yet implemented")
}
override fun onError(message: String?) {
TODO("Not yet implemented")
}
})
}
}
}<file_sep>/AdMediator/src/main/java/com/nezamipour/mehdi/admediator/mainclasses/companies/CompanyManager.kt
package com.nezamipour.mehdi.admediator.mainclasses.companies
import android.app.Activity
import android.app.Application
import android.content.Context
import com.nezamipour.mehdi.admediator.mainclasses.listeners.AdRequestCallback
import com.nezamipour.mehdi.admediator.mainclasses.listeners.AdShowCallback
interface CompanyManager {
fun initial(application: Application, appId: String)
fun requestAd(context: Context, zoneId: String, adRequestCallback: AdRequestCallback)
fun showAd(activity: Activity,
zoneId: String?,
adId: String,
adShowCallback: AdShowCallback
)
}<file_sep>/AdMediator/src/main/java/com/nezamipour/mehdi/admediator/mainclasses/listeners/AdRequestCallback.kt
package com.nezamipour.mehdi.admediator.mainclasses.listeners
interface AdRequestCallback {
fun onSuccess(adId: String?)
fun error(message: String?)
}<file_sep>/AdMediator/src/main/java/com/nezamipour/mehdi/admediator/network/ApiService.kt
package com.nezamipour.mehdi.admediator.network
import com.nezamipour.mehdi.admediator.model.AdConfig
import com.nezamipour.mehdi.admediator.model.AppConfig
import retrofit2.Response
import retrofit2.http.GET
import retrofit2.http.Query
interface ApiService {
// fake api
// base url : https://api.admediator.com/
// path : mobile_ad
@GET(NetworkConstants.MOBILE_AD)
suspend fun getAppConfig(@Query("appId") appId: String): Response<AppConfig>
@GET(NetworkConstants.MOBILE_AD)
suspend fun getAdConfig(@Query("zoneId") zoneId: String): Response<AdConfig>
}<file_sep>/AdMediator/src/main/java/com/nezamipour/mehdi/admediator/mainclasses/companies/TapSellManager.kt
package com.nezamipour.mehdi.admediator.mainclasses.companies
import android.app.Activity
import android.app.Application
import android.content.Context
import com.nezamipour.mehdi.admediator.mainclasses.AdMediator
import com.nezamipour.mehdi.admediator.mainclasses.listeners.AdRequestCallback
import com.nezamipour.mehdi.admediator.mainclasses.listeners.AdShowCallback
import ir.tapsell.sdk.Tapsell
import ir.tapsell.sdk.TapsellAdRequestListener
import ir.tapsell.sdk.TapsellAdShowListener
object TapSellManager : CompanyManager {
override fun initial(application: Application, appId: String) {
Tapsell.initialize(application, appId)
}
override fun requestAd(context: Context, zoneId: String, adRequestCallback: AdRequestCallback) {
Tapsell.requestAd(context, zoneId, null, object : TapsellAdRequestListener() {
override fun onAdAvailable(adId: String) {
super.onAdAvailable(adId)
adRequestCallback.onSuccess(adId)
AdMediator.sAdId = adId
AdMediator.adFound = true
}
override fun onError(message: String) {
super.onError(message)
AdMediator.adFound = false
}
})
}
override fun showAd(
activity: Activity,
zoneId: String?,
adId: String,
adShowCallback: AdShowCallback
) {
Tapsell.showAd(activity, zoneId, adId, null, object : TapsellAdShowListener() {
override fun onOpened() {
super.onOpened()
adShowCallback.onOpened()
}
override fun onError(s: String) {
super.onError(s)
adShowCallback.onError(s)
}
})
}
}<file_sep>/AdMediator/src/main/java/com/nezamipour/mehdi/admediator/mainclasses/companies/UnityAdsManager.kt
package com.nezamipour.mehdi.admediator.mainclasses.companies
import android.app.Activity
import android.app.Application
import android.content.Context
import com.nezamipour.mehdi.admediator.mainclasses.AdMediator
import com.nezamipour.mehdi.admediator.mainclasses.listeners.AdRequestCallback
import com.nezamipour.mehdi.admediator.mainclasses.listeners.AdShowCallback
import com.nezamipour.mehdi.admediator.mainclasses.listeners.UnityAdListeners
import com.unity3d.ads.IUnityAdsLoadListener
import com.unity3d.ads.UnityAds
object UnityAdsManager : CompanyManager {
override fun initial(application: Application, appId: String) {
UnityAds.initialize(application, appId)
}
override fun requestAd(context: Context, zoneId: String, adRequestCallback: AdRequestCallback) {
UnityAds.load(zoneId, object : IUnityAdsLoadListener {
override fun onUnityAdsAdLoaded(adId: String) {
adRequestCallback.onSuccess(adId)
AdMediator.adFound = true
AdMediator.sAdId = adId
}
override fun onUnityAdsFailedToLoad(placementId: String) {
adRequestCallback.error("placementId : $placementId")
AdMediator.adFound = false
}
})
}
override fun showAd(
activity: Activity,
zoneId: String?,
adId: String,
adShowCallback: AdShowCallback
) {
UnityAds.addListener(object : UnityAdListeners() {
override fun onUnityAdsStart(placementId: String?) {
super.onUnityAdsStart(placementId)
adShowCallback.onOpened()
}
override fun onUnityAdsError(error: UnityAds.UnityAdsError?, message: String?) {
super.onUnityAdsError(error, message)
adShowCallback.onError(message)
}
})
if (UnityAds.isReady(zoneId))
UnityAds.show(activity, zoneId)
}
}<file_sep>/AdMediator/src/main/java/com/nezamipour/mehdi/admediator/mainclasses/companies/ChartBoostManager.kt
package com.nezamipour.mehdi.admediator.mainclasses.companies
import android.app.Activity
import android.app.Application
import android.content.Context
import com.chartboost.sdk.Chartboost
import com.chartboost.sdk.ChartboostDelegate
import com.chartboost.sdk.Model.CBError
import com.nezamipour.mehdi.admediator.mainclasses.AdMediator
import com.nezamipour.mehdi.admediator.mainclasses.listeners.AdRequestCallback
import com.nezamipour.mehdi.admediator.mainclasses.listeners.AdShowCallback
import com.nezamipour.mehdi.admediator.utils.Constants
object ChartBoostManager : CompanyManager {
override fun initial(application: Application, appId: String) {
Chartboost.startWithAppId(application, appId, null)
}
override fun requestAd(context: Context, zoneId: String, adRequestCallback: AdRequestCallback) {
when (AdMediator.zoneType) {
Constants.AD_MEDIATOR_INTERSTITIAL_BANNER, Constants.AD_MEDIATOR_INTERSTITIAL_VIDEO -> requestInterstitialFromChartBoost(
zoneId, adRequestCallback
)
Constants.AD_MEDIATOR_REWARDED_VIDEO -> requestRewardedFromChartBoost(
zoneId,
adRequestCallback
)
}
}
private fun requestInterstitialFromChartBoost(
chartBoostZoneId: String,
adRequestCallback: AdRequestCallback
) {
Chartboost.setDelegate(object : ChartboostDelegate() {
override fun didCacheInterstitial(location: String?) {
super.didCacheInterstitial(location)
adRequestCallback.onSuccess(location)
}
override fun didFailToLoadInterstitial(
location: String?,
error: CBError.CBImpressionError?
) {
super.didFailToLoadInterstitial(location, error)
adRequestCallback.error(error.toString())
}
})
Chartboost.cacheInterstitial(chartBoostZoneId)
}
private fun requestRewardedFromChartBoost(
chartBoostZoneId: String,
adRequestCallback: AdRequestCallback
) {
Chartboost.setDelegate(object : ChartboostDelegate() {
override fun didCacheRewardedVideo(location: String?) {
super.didCacheRewardedVideo(location)
adRequestCallback.onSuccess(location)
}
override fun didFailToLoadRewardedVideo(
location: String?,
error: CBError.CBImpressionError?
) {
super.didFailToLoadRewardedVideo(location, error)
adRequestCallback.error(error.toString())
}
})
Chartboost.cacheRewardedVideo(chartBoostZoneId)
}
override fun showAd(
activity: Activity,
zoneId: String?,
adId: String,
adShowCallback: AdShowCallback
) {
when (AdMediator.zoneType) {
Constants.AD_MEDIATOR_INTERSTITIAL_BANNER, Constants.AD_MEDIATOR_INTERSTITIAL_VIDEO -> showInterstitialFromChartBoost(
zoneId, adShowCallback
)
Constants.AD_MEDIATOR_REWARDED_VIDEO -> showRewardedFromChartBoost(
zoneId,
adShowCallback
)
}
}
private fun showInterstitialFromChartBoost(
zoneId: String?, adShowCallback: AdShowCallback
) {
Chartboost.setDelegate(object : ChartboostDelegate() {
override fun didCloseInterstitial(location: String?) {
super.didCloseInterstitial(location)
adShowCallback.onClosed()
}
override fun didDisplayInterstitial(location: String?) {
super.didDisplayInterstitial(location)
adShowCallback.onOpened()
}
})
Chartboost.showInterstitial(zoneId)
}
private fun showRewardedFromChartBoost(
zoneId: String?, adShowCallback: AdShowCallback
) {
Chartboost.setDelegate(object : ChartboostDelegate() {
override fun didCloseRewardedVideo(location: String?) {
super.didCloseRewardedVideo(location)
adShowCallback.onClosed()
}
override fun didClickRewardedVideo(location: String?) {
super.didClickRewardedVideo(location)
}
override fun didCompleteRewardedVideo(location: String?, reward: Int) {
super.didCompleteRewardedVideo(location, reward)
adShowCallback.onRewarded()
}
override fun didDisplayRewardedVideo(location: String?) {
super.didDisplayRewardedVideo(location)
adShowCallback.onOpened()
}
})
Chartboost.showRewardedVideo(zoneId)
}
}<file_sep>/AdMediator/src/main/java/com/nezamipour/mehdi/admediator/model/AppConfig.kt
package com.nezamipour.mehdi.admediator.model
import android.os.Parcelable
import com.google.gson.annotations.Expose
import com.google.gson.annotations.SerializedName
import kotlinx.parcelize.Parcelize
@Parcelize
data class AppConfig(
@SerializedName("adNetworks")
@Expose
var adNetworks: AdNetworks
): Parcelable<file_sep>/AdMediator/src/main/java/com/nezamipour/mehdi/admediator/model/Waterfall.kt
package com.nezamipour.mehdi.admediator.model
import android.os.Parcelable
import com.google.gson.annotations.Expose
import com.google.gson.annotations.SerializedName
import kotlinx.parcelize.Parcelize
@Parcelize
data class Waterfall(
@SerializedName("adNetwork")
@Expose
var adNetwork: String,
@SerializedName("zoneId")
@Expose
var zoneId: String,
@SerializedName("timeout")
@Expose
var timeout: Long
) : Parcelable<file_sep>/AdMediator/src/main/java/com/nezamipour/mehdi/admediator/model/AdConfig.kt
package com.nezamipour.mehdi.admediator.model
import android.os.Parcelable
import com.google.gson.annotations.Expose
import com.google.gson.annotations.SerializedName
import kotlinx.parcelize.Parcelize
@Parcelize
data class AdConfig(
@SerializedName("zoneType")
@Expose
var zoneType: String,
@SerializedName("waterfall")
@Expose
var waterfall: List<Waterfall>,
@SerializedName("ttl")
@Expose
var ttl: Long
) : Parcelable<file_sep>/app/src/main/java/com/nezamipour/mehdi/tapselltaskwithkotlin/InterstitialVideoActivity.kt
package com.nezamipour.mehdi.tapselltaskwithkotlin
import android.content.Context
import android.content.Intent
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
class InterstitialVideoActivity : AppCompatActivity() {
companion object {
fun newIntent(context: Context): Intent {
return Intent(context, InterstitialVideoActivity::class.java)
}
}
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_interstitial_video)
}
}<file_sep>/AdMediator/src/main/java/com/nezamipour/mehdi/admediator/network/RetrofitInstance.kt
package com.nezamipour.mehdi.admediator.network
import com.jakewharton.retrofit2.adapter.kotlin.coroutines.CoroutineCallAdapterFactory
import retrofit2.Retrofit
import retrofit2.converter.gson.GsonConverterFactory
object RetrofitInstance {
val retrofitInstance = Retrofit.Builder()
.baseUrl(NetworkConstants.BASE_URL)
.addConverterFactory(GsonConverterFactory.create())
.addCallAdapterFactory(CoroutineCallAdapterFactory())
.build()
}<file_sep>/AdMediator/src/main/java/com/nezamipour/mehdi/admediator/network/NetworkConstants.kt
package com.nezamipour.mehdi.admediator.network
class NetworkConstants {
companion object {
const val BASE_URL = "https://api.admediator.com/"
const val MOBILE_AD = "mobile_ad"
}
}<file_sep>/app/src/main/java/com/nezamipour/mehdi/tapselltaskwithkotlin/App.kt
package com.nezamipour.mehdi.tapselltaskwithkotlin
import android.app.Application
import com.nezamipour.mehdi.admediator.mainclasses.AdMediator
class App : Application() {
override fun onCreate() {
super.onCreate()
AdMediator.initialize(this, "AppId")
}
}<file_sep>/AdMediator/src/main/java/com/nezamipour/mehdi/admediator/model/AdNetworks.kt
package com.nezamipour.mehdi.admediator.model
import android.os.Parcelable
import com.google.gson.annotations.Expose
import com.google.gson.annotations.SerializedName
import kotlinx.parcelize.Parcelize
@Parcelize
class AdNetworks(
@SerializedName("Tapsell")
@Expose
var tapsell: String?,
@SerializedName("UnityAds")
@Expose
var unityAds: String?,
@SerializedName("Chartboost")
@Expose
var chartboost: String?
): Parcelable<file_sep>/app/src/main/java/com/nezamipour/mehdi/tapselltaskwithkotlin/MainActivity.kt
package com.nezamipour.mehdi.tapselltaskwithkotlin
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import com.nezamipour.mehdi.admediator.mainclasses.AdMediator
import com.nezamipour.mehdi.tapselltaskwithkotlin.databinding.ActivityMainBinding
class MainActivity : AppCompatActivity() {
private lateinit var binding: ActivityMainBinding
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = ActivityMainBinding.inflate(layoutInflater)
setContentView(binding.root)
binding.buttonRewardedVideo.setOnClickListener {
startActivity(RewardedVideoActivity.newIntent(this))
}
binding.buttonInterstitialBanner.setOnClickListener {
startActivity(InterstitialBannerActivity.newIntent(this))
}
binding.buttonInterstitialVideo.setOnClickListener {
startActivity(InterstitialVideoActivity.newIntent(this))
}
}
}<file_sep>/AdMediator/src/main/java/com/nezamipour/mehdi/admediator/utils/Constants.kt
package com.nezamipour.mehdi.admediator.utils
class Constants {
companion object {
// Our Mediator
const val AD_MEDIATOR_INTERSTITIAL_BANNER = "INTERSTITIAL_BANNER_ZONE_ID"
const val AD_MEDIATOR_INTERSTITIAL_VIDEO = "INTERSTITIAL_VIDEO_ZONE_ID"
const val AD_MEDIATOR_REWARDED_VIDEO = "REWARDED_VIDEO_ZONE_ID"
//TapSell
const val TAPSELL_KEY =
"<KEY>"
const val TAPSELL_INTERSTITIAL_BANNER = "5caae7c33a2e170001ef9392"
const val TAPSELL_INTERSTITIAL_VIDEO = "5caaeffec1ed8b000149cedc"
const val TAPSELL_REWARDED_VIDEO = "5caaf03dc1ed8b000149cedd"
//UnityAd
const val UNITY_AD_INTERSTITIAL_BANNER = "UNITY_AD_INTERSTITIAL_BANNER"
const val UNITY_AD_INTERSTITIAL_VIDEO = "UNITY_AD_INTERSTITIAL_VIDEO"
const val UNITY_AD_REWARDED_VIDEO = "UNITY_AD_REWARDED_VIDEO"
//Chartboost
const val CHART_BOOST_INTERSTITIAL_BANNER = "CHART_BOOST_INTERSTITIAL_BANNER"
const val CHART_BOOST_INTERSTITIAL_VIDEO = "CHART_BOOST_INTERSTITIAL_VIDEO"
const val CHART_BOOST_REWARDED_VIDEO = "CHART_BOOST_REWARDED_VIDEO"
const val APP_CONFIG = "{\n" +
"\"adNetworks\" : {\n" +
"\"Tapsell\" : \"appIdInTapsell\",\n" +
"\"UnityAds\" : \"appIdInUnityAds\",\n" +
"\"Chartboost\" : \"appIdInChartboost\"\n" +
"}\n" +
"}"
const val AD_CONFIG = "{\n" +
"\"zoneType\": \"Interstitial\",\n" +
"\"waterfall\": [\n" +
"{\n" +
"\"adNetwork\": \"UnityAds\",\n" +
"\"zoneId\": \"zoneIdInUnityAds\",\n" +
"\"timeout\": 2000\n" +
"},\n" +
"{\n" +
"\"adNetwork\": \"Tapsell\",\n" +
"\"zoneId\": \"zoneIdInTapsell\",\n" +
"\"timeout\": 3000\n" +
"},\n" +
"{\n" +
"\"adNetwork\": \"Chartboost\",\n" +
"\"zoneId\": \"zoneIdInChartboost\",\n" +
"\"timeout\": 1000\n" +
"}\n" +
"],\n" +
"\"ttl\": 3600000\n" +
"}"
}
}<file_sep>/settings.gradle
include ':AdMediator'
include ':app'
rootProject.name = "TapsellTaskWithKotlin"<file_sep>/README.md
# TapsellTaskWithKotlin
Simple Ad Mediator Library
# Add dependency
add these to gradle (project):
maven { url 'https://jitpack.io' }
<br>
maven {url 'https://dl.bintray.com/tapsellorg/maven'}
<br>
maven { url "https://chartboostmobile.bintray.com/Chartboost" }
then add these dependencies to app module:
implementation 'com.github.MehdiNezamipour:TapsellTaskWithKotlin:v.1.0'
# Guide of usage
call static methods on AdMediator class like initialize, adRequest, showAd.
<file_sep>/AdMediator/src/main/java/com/nezamipour/mehdi/admediator/network/AdMediatorService.kt
package com.nezamipour.mehdi.admediator.network
import com.nezamipour.mehdi.admediator.model.AdConfig
import com.nezamipour.mehdi.admediator.model.AppConfig
object AdMediatorService : BaseService() {
private val apiService = RetrofitInstance.retrofitInstance.create(ApiService::class.java)
suspend fun getAppConfig(appId: String): AppConfig {
when (val result = createCall { apiService.getAppConfig(appId) }) {
is Result.Success -> result.data
is Result.Error -> result.throwable
}
throw Throwable("getAppConfig : not work")
}
suspend fun getAdConfig(zoneId: String): AdConfig {
when (val result = createCall { apiService.getAdConfig(zoneId) }) {
is Result.Success -> result.data
is Result.Error -> result.throwable
}
throw Throwable("getAdConfig : not work")
}
}<file_sep>/AdMediator/src/main/java/com/nezamipour/mehdi/admediator/mainclasses/listeners/UnityAdListeners.kt
package com.nezamipour.mehdi.admediator.mainclasses.listeners
import com.unity3d.ads.IUnityAdsListener
import com.unity3d.ads.UnityAds.FinishState
import com.unity3d.ads.UnityAds.UnityAdsError
open class UnityAdListeners : IUnityAdsListener {
override fun onUnityAdsReady(placementId: String?) {}
override fun onUnityAdsStart(placementId: String?) {}
override fun onUnityAdsFinish(placementId: String?, result: FinishState?) {}
override fun onUnityAdsError(error: UnityAdsError?, message: String?) {}
}<file_sep>/AdMediator/src/main/java/com/nezamipour/mehdi/admediator/mainclasses/listeners/AdShowCallback.kt
package com.nezamipour.mehdi.admediator.mainclasses.listeners
interface AdShowCallback {
fun onOpened()
fun onClosed()
fun onRewarded()
fun onError(message: String?)
} | e13430ceb16a1f9e8f11b274878ac066a3029629 | [
"Markdown",
"Kotlin",
"Gradle"
] | 23 | Kotlin | MehdiNezamipour/TapsellTaskWithKotlin | 6128b5434f19f07b940b235aabfb5f2f833e0e1e | 571d3cd621a0e081233a0ac9d75dfa1974fa2e9b | |
refs/heads/master | <file_sep># Doctrine Extensions Rateable
This repository contains the rateable extension for Doctrine 2. This allows to
rate your doctrine entities easily.
## Use
Each rate is a tuple consisting of a resource id, a user id and a score.
### Implement the DoctrineExtensions\Rateable\Rateable interface.
First, your entity must implement the `DoctrineExtensions\Rateable\Rateable` interface.
Five methods in your entity must be written:
* `getRateableId()`
* `getRateableType()`
* `getRatingVotes()`
* `setRatingVotes($number)`
* `getRatingTotal()`
* `setRatingTotal($number)`
Example:
namespace MyProject;
use DoctrineExtensions\Rateable\Rateable;
/**
* @Entity
*/
class Article implements Rateable
{
/**
* @Id
* @GeneratedValue
* @Column(type="integer")
*/
protected $id;
/**
* @Column(name="rating_votes", type="integer")
*/
protected $ratingVotes = 0;
/**
* @Column(name="rating_total", type="integer")
*/
protected $ratingTotal = 0;
public function getId()
{
return $this->id;
}
public function getResourceId()
{
return 'article-'.$this->getId();
}
public function getRatingVotes()
{
return $this->ratingVotes;
}
public function setRatingVotes($number)
{
$this->ratingVotes = $number;
}
public function getRatingTotal()
{
return $this->ratingTotal;
}
public function setRatingTotal($number)
{
$this->ratingTotal = $number;
}
}
### Implement the DoctrineExtensions\Rateable\Reviewer interface.
Second, your user entity must implement `DoctrineExtensions\Rateable\Reviewer` interface.
Four methods are needed:
* `getReviewerId()`
* `canAddRate(Rateable $resource)`
* `canChangeRate(Rateable $resource)`
* `canRemoveRate(Rateable $resource)`
Example:
namespace MyProject;
use DoctrineExtensions\Rateable\Rateable;
use DoctrineExtensions\Rateable\Reviewer;
/**
* @Entity
*/
class User implements Reviewer
{
/**
* @Id
* @GeneratedValue
* @Column(type="integer")
*/
protected $id;
public function getId()
{
return $this->id;
}
public function getReviewerId()
{
return $this->getId();
}
public function canAddRate(Rateable $resource)
{
return true;
}
public function canChangeRate(Rateable $resource)
{
return true;
}
public function canRemoveRate(Rateable $resource)
{
return false;
}
}
### Setup Doctrine
Finally, you need to setup doctrine for register metadata directory and register RatingListener.
First, register the metadata directory of this package.
$config = new \Doctrine\ORM\Configuration();
// ...
$driverImpl = new \Doctrine\ORM\Mapping\Driver\XmlDriver(array('/path/to/doctrine-extensions-rateable/metadata'));
$config->setMetadataDriverImpl($driverImpl);
or with `DriverChain`:
$driverImpl = new \Doctrine\ORM\Mapping\Driver\DriverChain();
// ...
$driverImpl->addDriver(new \Doctrine\ORM\Mapping\Driver\XmlDriver('/path/to/doctrine-extensions-rateable/metadata'), 'DoctrineExtensions\\Rateable\\Entity');
Then, register the RatingListener.
// $this->em = EntityManager::create($connection, $config);
// ...
$this->ratingManager = new RatingManager($this->em);
$this->em->getEventManager()->addEventSubscriber(new RatingListener($this->ratingManager));
### Using RatingManager
Now, you can use RatingManager.
// Add a new rate..
$this->ratingManager->addRate($resource, $user, 4);
// Change my rate..
$this->ratingManager->changeRate($resource, $user, 2);
// Remove my rate..
try {
$this->ratingManager->removeRate($resource, $user);
} catch (PermissionDeniedException $e) {
echo 'Oh, no permission to remove my rate!';
}
// Compute resource rating score...
$this->ratingManager->getRatingScore($resource); // will return 2
### Exceptions
Rateable extension can throw four different exceptions:
* `InvalidRateScoreException` when your rate score is not between 1 and 5
* `NotFoundRateException` when your try to change or remove an non existant rate
* `PermissionDeniedException` when user have not permission to do this action
* `ResourceAlreadyRatedException` when user have already rate the resource and you try to add new one
<file_sep><?php
/*
* This file is part of the Doctrine Extensions Rateable package.
* (c) 2011 <NAME> <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace DoctrineExtensions\Rateable\Exception;
class InvalidRateScoreException extends \Exception
{
public function __construct($minValue, $maxValue, $code = null)
{
parent::__construct(
sprintf('Rate score must be between %d and %d', $minValue, $maxValue),
$code
);
}
}
<file_sep><?php
/*
* This file is part of the Doctrine Extensions Rateable package.
* (c) 2011 <NAME> <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace DoctrineExtensions\Rateable;
/**
* Reviewer is the interface that user entity must implement.
*
* @author <NAME> <<EMAIL>>
*/
interface Reviewer
{
/**
* Returns unique identifiant
*
* @return integer An unique identifiant
*/
function getReviewerId();
/**
* Check if user can add a rate for the resource
*
* @return Boolean TRUE if user can add a rate, FALSE otherwise.
*/
function canAddRate(Rateable $resource);
/**
* Check if user can change his rate for the resource
*
* @return Boolean TRUE if user can change his rate, FALSE otherwise.
*/
function canChangeRate(Rateable $resource);
/**
* Check if user can remove his rate for the resource
*
* @return Boolean TRUE if user can remove his rate, FALSE otherwise.
*/
function canRemoveRate(Rateable $resource);
}
<file_sep><?php
namespace Tests\DoctrineExtensions\Rateable\Fixtures;
use DoctrineExtensions\Rateable\Rateable;
use DoctrineExtensions\Rateable\Reviewer;
/**
* @Entity
*/
class User implements Reviewer
{
/**
* @Id
* @GeneratedValue
* @Column(type="integer")
*/
public $id = 0;
/**
* @Column(name="username", type="string", length=50)
*/
public $username;
public $canAddRate = true;
public $canChangeRate = true;
public $canRemoveRate = true;
public function getId()
{
return $this->id;
}
public function getReviewerId()
{
return $this->getId();
}
public function canAddRate(Rateable $resource)
{
return $this->canAddRate;
}
public function canChangeRate(Rateable $resource)
{
return $this->canChangeRate;
}
public function canRemoveRate(Rateable $resource)
{
return $this->canRemoveRate;
}
}
<file_sep><?php
namespace Tests\DoctrineExtensions\Rateable\Fixtures;
use DoctrineExtensions\Rateable\Entity\Rate as BaseRate;
/**
* @Entity
*/
class Rate extends BaseRate
{
}
<file_sep><?php
/*
* This file is part of the Doctrine Extensions Rateable package.
* (c) 2011 <NAME> <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
require_once __DIR__.'/Fixtures/Article.php';
require_once __DIR__.'/Fixtures/User.php';
require_once __DIR__.'/Fixtures/Rate.php';
use DoctrineExtensions\Rateable\RatingManager;
use DoctrineExtensions\Rateable\RatingListener;
use Tests\DoctrineExtensions\Rateable\Fixtures\User;
use Tests\DoctrineExtensions\Rateable\Fixtures\Article;
class RatingManagerTest extends \PHPUnit_Framework_TestCase
{
protected $em;
protected $manager;
protected $article;
public function setUp()
{
$config = new \Doctrine\ORM\Configuration();
$config->setMetadataCacheImpl(new \Doctrine\Common\Cache\ArrayCache);
$config->setQueryCacheImpl(new \Doctrine\Common\Cache\ArrayCache);
$config->setProxyDir(__DIR__ . '/Proxy');
$config->setProxyNamespace('DoctrineExtensions\Rateable\Proxies');
//$config->setMetadataDriverImpl($config->newDefaultAnnotationDriver());
$driverImpl = new \Doctrine\ORM\Mapping\Driver\DriverChain();
$driverImpl->addDriver(new \Doctrine\ORM\Mapping\Driver\XmlDriver(__DIR__.'/../../../metadata'), 'DoctrineExtensions\\Rateable\\Entity');
$driverImpl->addDriver($config->newDefaultAnnotationDriver(), 'Tests\\DoctrineExtensions\\Rateable\\Fixtures');
$config->setMetadataDriverImpl($driverImpl);
$this->em = \Doctrine\ORM\EntityManager::create(
array('driver' => 'pdo_sqlite', 'memory' => true),
$config
);
$schemaTool = new \Doctrine\ORM\Tools\SchemaTool($this->em);
$schemaTool->dropSchema(array());
$schemaTool->createSchema(array(
$this->em->getClassMetadata('DoctrineExtensions\\Rateable\\Entity\\Rate'),
$this->em->getClassMetadata('Tests\\DoctrineExtensions\\Rateable\\Fixtures\\Article'),
$this->em->getClassMetadata('Tests\\DoctrineExtensions\\Rateable\\Fixtures\\User'),
));
$article1 = new Article();
$article1->title = 'Unit Testing';
$article1->body = 'My first article about unit testing';
$this->em->persist($article1);
$this->article = new Article();
$this->article->title = 'Unit Testing #2';
$this->article->body = 'My second article about unit testing';
$this->em->persist($this->article);
$user1 = new User();
$user1->username = 'Fabien';
$this->em->persist($user1);
$user2 = new User();
$user2->username = 'Fabien2';
$this->em->persist($user2);
$this->em->flush();
$this->manager = new RatingManager($this->em);
$this->em->getEventManager()->addEventSubscriber(new RatingListener($this->manager));
$this->manager->addRate($article1, $user1, 5);
$this->manager->addRate($this->article, $user1, 3);
$this->manager->addRate($this->article, $user2, 2);
}
public function testConstructor()
{
$manager = new RatingManager($this->em);
$this->assertInstanceOf('DoctrineExtensions\Rateable\RatingManager', $manager);
$class = 'Tests\DoctrineExtensions\Rateable\Fixtures\Rate';
$manager = new RatingManager($this->em, $class);
$this->assertEquals($class, $manager->getRateClass());
}
public function testSetMultiRating()
{
$this->manager->setMultiRating(true);
$this->assertEquals(true, $this->manager->getMultiRating());
$this->assertEquals(86400, $this->manager->getTimeBeforeNewRating());
$this->manager->setMultiRating(false);
$this->assertEquals(false, $this->manager->getMultiRating());
$this->assertEquals(86400, $this->manager->getTimeBeforeNewRating());
$this->manager->setMultiRating(true, 3600);
$this->assertEquals(3600, $this->manager->getTimeBeforeNewRating());
}
public function testAddRate()
{
$user = new User();
$user->id = 123;
$this->assertEquals(2, $this->article->getRatingVotes());
$this->assertEquals(5, $this->article->getRatingTotal());
$this->manager->addRate($this->article, $user, 4);
$this->assertEquals(3, $this->article->getRatingVotes());
$this->assertEquals(9, $this->article->getRatingTotal());
$rate = $this->manager->findRate($this->article, $user);
$this->assertEquals($this->article->getRateableId(), $rate->getResourceId());
$this->assertEquals($this->article->getRateableType(), $rate->getResourceType());
$this->assertEquals(4, $rate->getScore());
}
/**
* @expectedException DoctrineExtensions\Rateable\Exception\PermissionDeniedException
*/
public function testAddRateWithoutPermission()
{
$user = new User();
$user->id = 456;
$user->canAddRate = false;
$this->manager->addRate($this->article, $user, 2);
}
/**
* @expectedException DoctrineExtensions\Rateable\Exception\InvalidRateScoreException
*/
public function testAddRateWithScoreLessThanOne()
{
$this->manager->addRate(new Article(), new User(), 0);
}
/**
* @expectedException DoctrineExtensions\Rateable\Exception\InvalidRateScoreException
*/
public function testAddRateWithScoreMoreThanFive()
{
$this->manager->addRate(new Article(), new User(), 6);
}
/**
* @expectedException DoctrineExtensions\Rateable\Exception\ResourceAlreadyRatedException
*/
public function testAddRateAgain()
{
$user = new User();
$user->id = 123;
$this->manager->addRate($this->article, $user, 4);
$this->manager->addRate($this->article, $user, 4);
}
public function testAddRateAgainWithMultiRatingNoWait()
{
$user = new User();
$user->id = 951;
// First Test unlimited re-rate (no wait)
$this->manager->setMultiRating(true, 0);
$this->manager->addRate($this->article, $user, 3);
$this->assertEquals(3, $this->article->getRatingVotes());
$this->manager->addRate($this->article, $user, 3);
$this->assertEquals(4, $this->article->getRatingVotes());
}
/**
* @expectedException DoctrineExtensions\Rateable\Exception\ResourceAlreadyRatedInPeriodException
*/
public function testAddRateAgainWithMultiRatingWait()
{
$user = new User();
$user->id = 951;
// Then Test Mandatory waiting fail
$this->manager->setMultiRating(true, 3600);
$this->manager->addRate($this->article, $user, 3);
$this->manager->addRate($this->article, $user, 3);
}
public function testAddRateAgainWithMultiRatingWaitOk()
{
$user = new User();
$user->id = 851;
// Then Test Mandatory waiting
$this->manager->setMultiRating(true, 3600);
$rate = $this->manager->addRate($this->article, $user, 3);
$rate->setCreatedAt(new \DateTime('now-1 day'));
$this->em->persist($rate);
$this->em->flush();
$this->manager->addRate($this->article, $user, 3);
}
public function testChangeRate()
{
$user = new User();
$user->id = 123;
$this->manager->addRate($this->article, $user, 4);
$this->assertEquals(4, $this->manager->findRate($this->article, $user)->getScore());
$this->assertEquals(9, $this->article->getRatingTotal());
sleep(1);
$this->manager->changeRate($this->article, $user, 2);
$this->assertEquals(3, $this->article->getRatingVotes());
$this->assertEquals(7, $this->article->getRatingTotal());
$rate = $this->manager->findRate($this->article, $user);
$this->assertEquals(2, $rate->getScore());
$this->assertEquals(new \DateTime('now'), $rate->getUpdatedAt());
}
/**
* @expectedException DoctrineExtensions\Rateable\Exception\PermissionDeniedException
*/
public function testChangeRateWithoutPermission()
{
$user = new User();
$user->id = 456;
$user->canChangeRate = false;
$this->manager->changeRate($this->article, $user, 2);
}
/**
* @expectedException DoctrineExtensions\Rateable\Exception\InvalidRateScoreException
*/
public function testChangeRateWithScoreLessThanOne()
{
$this->manager->changeRate(new Article(), new User(), 0);
}
/**
* @expectedException DoctrineExtensions\Rateable\Exception\InvalidRateScoreException
*/
public function testChangeRateWithScoreMoreThanFive()
{
$this->manager->changeRate(new Article(), new User(), 6);
}
/**
* @expectedException DoctrineExtensions\Rateable\Exception\NotFoundRateException
*/
public function testChangeRateWithInvalidRate()
{
$user = new User();
$user->id = 123;
$article = new Article();
$article->id = 456;
$this->manager->changeRate($article, $user, 2);
}
public function testRemoveRate()
{
$user = new User();
$user->id = 123;
$this->manager->addRate($this->article, $user, 4);
$this->assertEquals(4, $this->manager->findRate($this->article, $user)->getScore());
$this->assertEquals(3, $this->article->getRatingVotes());
$this->manager->removeRate($this->article, $user);
$this->assertNull($this->manager->findRate($this->article, $user));
$this->assertEquals(2, $this->article->getRatingVotes());
$this->assertEquals(5, $this->article->getRatingTotal());
}
/**
* @expectedException DoctrineExtensions\Rateable\Exception\PermissionDeniedException
*/
public function testRemoveRateWithoutPermission()
{
$user = new User();
$user->id = 456;
$user->canRemoveRate = false;
$this->manager->removeRate($this->article, $user, 2);
}
/**
* @expectedException DoctrineExtensions\Rateable\Exception\NotFoundRateException
*/
public function testRemoveRateWithInvalidRate()
{
$user = new User();
$user->id = 123;
$article = new Article();
$article->id = 456;
$this->manager->removeRate($article, $user);
}
public function testComputeRating()
{
$this->article->ratingVotes = 10;
$this->article->ratingTotal = 25;
$this->manager->computeRating($this->article);
$this->assertEquals(2, $this->article->getRatingVotes());
$this->assertEquals(5, $this->article->getRatingTotal());
}
public function testGetRatingScore()
{
$this->assertEquals(3., $this->manager->getRatingScore($this->article));
$this->assertEquals(2.5, $this->manager->getRatingScore($this->article, 1));
$this->article->ratingVotes = 0;
$this->article->ratingTotal = 0;
$this->assertEquals(0, $this->manager->getRatingScore($this->article));
}
public function testIsValidRateScore()
{
$this->assertTrue($this->manager->isValidRateScore(1));
$this->assertTrue($this->manager->isValidRateScore(5));
$this->assertFalse($this->manager->isValidRateScore(0));
$this->assertFalse($this->manager->isValidRateScore(6));
}
public function testDeleteResource()
{
$this->assertEquals(2, sizeof($this->manager->findRatesForResource($this->article)));
$article = clone $this->article;
$this->em->remove($this->article);
$this->em->flush();
$this->assertEquals(0, sizeof($this->manager->findRatesForResource($article)));
}
}
<file_sep><?php
/*
* This file is part of the Doctrine Extensions Rateable package.
* (c) 2011 <NAME> <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace DoctrineExtensions\Rateable;
use Doctrine\ORM\EntityManager;
/**
* RatingManager.
*
* @author <NAME> <<EMAIL>>
* @author Bat <<EMAIL>>
*/
class RatingManager
{
protected $em;
protected $minRateScore = 1;
protected $maxRateScore = 5;
/**
* Set if a user can add rating more than once
* @var boolean
*/
protected $multiRating = false;
/**
* Time before a user can re-rate. Only in case of multiRating.
* @var integer time in seconds
*/
protected $timeBeforeNewRating = 86400;
public function __construct(EntityManager $em, $class = null, $minRateScore = 1, $maxRateScore = 5)
{
$this->em = $em;
$this->class = $class ?: 'DoctrineExtensions\Rateable\Entity\Rate';
$this->minRateScore = $minRateScore;
$this->maxRateScore = $maxRateScore;
}
/**
* Set the permission to vote more than once
* @param boolean $multiRating
* @param integer $timeBeforeNewRating
*/
public function setMultiRating($multiRating, $timeBeforeNewRating = null)
{
$this->multiRating = $multiRating;
if (!is_null($timeBeforeNewRating)) $this->timeBeforeNewRating = $timeBeforeNewRating;
}
public function getMultiRating()
{
return $this->multiRating;
}
public function getTimeBeforeNewRating()
{
return $this->timeBeforeNewRating;
}
/**
* Adds a new rate.
*
* @param Rateable $resource The resource object
* @param Reviewer $reviewer The reviewer object
* @param integer $rateScore The rate score
*
* @return void
*/
public function addRate(Rateable $resource, Reviewer $reviewer, $rateScore, $save=true)
{
if (!$reviewer->canAddRate($resource)) {
throw new Exception\PermissionDeniedException('This reviewer cannot add a rate for this resource');
}
if (!$this->isValidRateScore($rateScore)) {
throw new Exception\InvalidRateScoreException($this->minRateScore, $this->maxRateScore);
}
if ((!$this->multiRating || $this->timeBeforeNewRating > 0) && $lastRate = $this->findRate($resource, $reviewer)) {
if (!$this->multiRating) {
throw new Exception\ResourceAlreadyRatedException('The reviewer has already rated this resource');
}
elseif( $lastRate->getCreatedAt()->getTimestamp() > time()-$this->getTimeBeforeNewRating()) {
throw new Exception\ResourceAlreadyRatedInPeriodException('The reviewer must wait before re-rate this resource');
}
}
$rate = $this->createRate();
$rate->setResource($resource);
$rate->setReviewer($reviewer);
$rate->setScore($rateScore);
$resource->setRatingVotes($resource->getRatingVotes() + 1);
$resource->setRatingTotal($resource->getRatingTotal() + $rateScore);
if ($save) {
$this->saveEntity($rate);
$this->saveEntity($resource);
}
return $rate;
}
/**
* Changes score for an existant rate.
* Warning : This method only works fine with single Rating
* @todo : add method to remove All Rate, or on Rate by id
*
* @param Rateable $resource The resource object
* @param Reviewer $reviewer The reviewer object
* @param integer $rateScore The new rate score
*
* @return void
*/
public function changeRate(Rateable $resource, Reviewer $reviewer, $rateScore)
{
if (!$reviewer->canChangeRate($resource)) {
throw new Exception\PermissionDeniedException('This reviewer cannot change his rate for this resource');
}
if (!$this->isValidRateScore($rateScore)) {
throw new Exception\InvalidRateScoreException($this->minRateScore, $this->maxRateScore);
}
if (!($rate = $this->findRate($resource, $reviewer))) {
throw new Exception\NotFoundRateException('Unable to find rate object');
}
$resource->setRatingTotal($resource->getRatingTotal() - $rate->getScore());
$resource->setRatingTotal($resource->getRatingTotal() + $rateScore);
$rate->setScore($rateScore);
$rate->setUpdatedAt(new \DateTime('now'));
$this->saveEntity($rate);
$this->saveEntity($resource);
}
/**
* Removes an existant rate.
* Warning : This method only works fine with single Rating
* @todo : add method to remove All Rate, or on Rate by id
*
* @param Rateable $resource The resource object
* @param Reviewer $reviewer The reviewer object
*
* @return void
*/
public function removeRate(Rateable $resource, Reviewer $reviewer)
{
if (!$reviewer->canRemoveRate($resource)) {
throw new Exception\PermissionDeniedException('This reviewer cannot remove his rate for this resource');
}
if (!($rate = $this->findRate($resource, $reviewer))) {
throw new Exception\NotFoundRateException('Unable to find rate object');
}
$resource->setRatingVotes($resource->getRatingVotes() - 1);
$resource->setRatingTotal($resource->getRatingTotal() - $rate->getScore());
$this->em->remove($rate);
$this->em->flush();
$this->saveEntity($resource);
}
/**
* Computes rating fields for an resource.
*
* @param Rateable $resource The resource object
*
* @return void
*/
public function computeRating(Rateable $resource)
{
$votes = 0;
$total = 0;
foreach ($this->findRatesForResource($resource) as $rate) {
$total += $rate->getScore();
$votes++;
}
$resource->setRatingVotes($votes);
$resource->setRatingTotal($total);
$this->saveEntity($resource);
}
/**
* Gets the rating score for a resource
*
* @param Rateable $resource The resource object
* @param integer $precision Score precision
*
* @return void
*/
public function getRatingScore(Rateable $resource, $precision=0)
{
if (!($resource->getRatingVotes() > 0)) {
return 0;
}
return round($resource->getRatingTotal() / $resource->getRatingVotes(), $precision);
}
/**
* @return integer min score allowed
*/
public function getMinRateScore()
{
return $this->minRateScore;
}
/**
* @return integer max score allowed
*/
public function getMaxScore()
{
return $this->maxRateScore;
}
/**
* @return array range of allowed scores
*/
public function getRange()
{
return range($this->minRateScore, $this->maxRateScore);
}
/**
* Finds the last rate object for a couple resource/reviewer.
*
* @param Rateable $resource The resource object
* @param Reviewer $reviewer The reviewer object
*
* @return null|Rate The rate object if exist, null otherwise
*/
public function findRate(Rateable $resource, Reviewer $reviewer)
{
return $this->em
->getRepository($this->class)
->findOneBy(array(
'resourceId' => $resource->getRateableId(),
'resourceType' => $resource->getRateableType(),
'reviewerId' => $reviewer->getReviewerId(),
), array('createdAt' => 'DESC'))
;
}
/**
* Finds rates collection for a couple resource/reviewer
*
* @param Rateable $resource
* @param Reviewer $reviewer
* @return DoctrineCollection
*/
public function findRates(Rateable $resource, Reviewer $reviewer)
{
return $this->em
->getRepository($this->class)
->findBy(array(
'resourceId' => $resource->getRateableId(),
'resourceType' => $resource->getRateableType(),
'reviewerId' => $reviewer->getReviewerId(),
), array('createdAt' => 'DESC'))
;
}
/**
* Finds all rate objects for a resource.
*
* @param Rateable $resource The resource object
*
* @return Doctrine\Common\Collection
*/
public function findRatesForResource(Rateable $resource)
{
return $this->em
->getRepository($this->class)
->findBy(array(
'resourceId' => $resource->getRateableId(),
'resourceType' => $resource->getRateableType(),
))
;
}
/**
* Checks if a value is a valid rate score
*
* @param integer $rateScore The rate score
*
* @return Boolean TRUE if value is valid, FALSE otherwise
*/
public function isValidRateScore($score)
{
return ($score >= $this->minRateScore
and $score <= $this->maxRateScore);
}
/**
* Returns the class name for Rate entity
*
* @return string
*/
public function getRateClass()
{
return $this->class;
}
/**
* Creates a new Rate object
*
* @return Rate
*/
protected function createRate()
{
return new $this->class();
}
/**
* Saves a Doctrine Entity
*
* @param mixed $entity The entity object
*
* @return mixed Entity object
*/
protected function saveEntity($entity)
{
$this->em->persist($entity);
$this->em->flush();
return $entity;
}
}
<file_sep><?php
/*
* This file is part of the Doctrine Extensions Rateable package.
* (c) 2011 <NAME> <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace DoctrineExtensions\Rateable\Exception;
class NotFoundRateException extends \Exception
{}
<file_sep><?php
namespace Tests\DoctrineExtensions\Rateable\Fixtures;
/**
* @Entity
*/
class Article implements \DoctrineExtensions\Rateable\Rateable
{
/**
* @Id
* @GeneratedValue
* @Column(type="integer")
*/
public $id = 0;
/**
* @Column(name="title", type="string", length=50)
*/
public $title;
/**
* @Column(name="body", type="string")
*/
public $body;
/**
* @Column(name="rating_votes", type="integer")
*/
public $ratingVotes = 0;
/**
* @Column(name="rating_total", type="integer")
*/
public $ratingTotal = 0;
public function getId()
{
return $this->id;
}
public function getRateableId()
{
return $this->getId();
}
public function getRateableType()
{
return 'Article';
}
public function getRatingVotes()
{
return $this->ratingVotes;
}
public function setRatingVotes($number)
{
$this->ratingVotes = $number;
}
public function getRatingTotal()
{
return $this->ratingTotal;
}
public function setRatingTotal($number)
{
$this->ratingTotal = $number;
}
}
<file_sep><?php
/*
* This file is part of the Doctrine Extensions Rateable package.
* (c) 2011 <NAME> <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace DoctrineExtensions\Rateable\Entity;
use DoctrineExtensions\Rateable\Rateable;
use DoctrineExtensions\Rateable\Reviewer;
class Rate
{
protected $id;
protected $resourceId;
protected $resourceType;
protected $reviewerId;
protected $score;
protected $createdAt;
protected $updatedAt;
public function __construct()
{
$this->createdAt = new \DateTime('now');
$this->updatedAt = new \DateTime('now');
}
public function getId()
{
return $this->id;
}
public function setResource(Rateable $resource)
{
$this->resourceId = $resource->getRateableId();
$this->resourceType = $resource->getRateableType();
}
public function getResourceId()
{
return $this->resourceId;
}
public function getResourceType()
{
return $this->resourceType;
}
public function setReviewer(Reviewer $reviewer)
{
$this->reviewerId = $reviewer->getReviewerId();
}
public function getReviewerId()
{
return $this->reviewerId;
}
public function setScore($score)
{
$this->score = $score;
}
public function getScore()
{
return $this->score;
}
public function setCreatedAt(\DateTime $date)
{
$this->createdAt = $date;
}
public function getCreatedAt()
{
return $this->createdAt;
}
public function setUpdatedAt(\DateTime $date)
{
$this->updatedAt = $date;
}
public function getUpdatedAt()
{
return $this->updatedAt;
}
}
<file_sep><?php
/*
* This file is part of the Doctrine Extensions Rateable package.
* (c) 2011 <NAME> <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace DoctrineExtensions\Rateable;
use Doctrine\Common\EventSubscriber;
use Doctrine\ORM\Events;
use Doctrine\ORM\Event\LifecycleEventArgs;
/**
* RatingListener.
*
* @author <NAME> <<EMAIL>>
*/
class RatingListener implements EventSubscriber
{
protected $manager;
/**
* Constructor
*
* @param ContainerInterface $container
*/
public function __construct(RatingManager $manager)
{
$this->manager = $manager;
}
/**
* @see Doctrine\Common\EventSubscriber
*/
public function getSubscribedEvents()
{
return array(Events::preRemove);
}
/**
* @param LifecycleEventArgs $args
*/
public function preRemove(LifecycleEventArgs $args)
{
if (($resource = $args->getEntity()) and $resource instanceof Rateable) {
$em = $args->getEntityManager();
foreach ($this->manager->findRatesForResource($resource) as $rate) {
$em->remove($rate);
}
}
}
}<file_sep><?php
/*
* This file is part of the Doctrine Extensions Rateable package.
* (c) 2011 <NAME> <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
require_once __DIR__.'/../Fixtures/Article.php';
require_once __DIR__.'/../Fixtures/User.php';
use DoctrineExtensions\Rateable\Rateable;
use DoctrineExtensions\Rateable\Entity\Rate;
use Tests\DoctrineExtensions\Rateable\Fixtures\User;
class RateTest extends \PHPUnit_Framework_TestCase
{
public function testConstructor()
{
$rate = new Rate();
$this->assertEquals(new \DateTime('now'), $rate->getCreatedAt());
$this->assertEquals(new \DateTime('now'), $rate->getUpdatedAt());
}
/**
* @covers DoctrineExtensions\Rateable\Entity\Rate::setResource
* @covers DoctrineExtensions\Rateable\Entity\Rate::getResourceId
*/
public function testSetGetResource()
{
$article = new \Tests\DoctrineExtensions\Rateable\Fixtures\Article();
$article->id = 123;
$rate = new Rate();
$rate->setResource($article);
$this->assertEquals($article->getRateableId(), $rate->getResourceId());
$this->assertEquals($article->getRateableType(), $rate->getResourceType());
}
/**
* @covers DoctrineExtensions\Rateable\Entity\Rate::setReviewer
* @covers DoctrineExtensions\Rateable\Entity\Rate::getReviewerId
*/
public function testSetGetReviewer()
{
$user = new User();
$user->id = 345;
$rate = new Rate();
$rate->setReviewer($user);
$this->assertEquals(345, $rate->getReviewerId());
}
}
<file_sep><?php
/*
* This file is part of the Doctrine Extensions Rateable package.
* (c) 2011 <NAME> <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace DoctrineExtensions\Rateable;
/**
* Rateable is the interface that rateable resource classes must implement.
*
* @author <NAME> <<EMAIL>>
* @author Bat <<EMAIL>>
*/
interface Rateable
{
/**
* Get rateable identifier, typically id (getId())
* return mixed
*/
function getRateableId();
/**
* Get rateable Type, typically class name
* @return string
*/
function getRateableType();
/**
* Return number of votes
* @return integer
*/
function getRatingVotes();
/**
* Set number of votes
* @param integer $number
*/
function setRatingVotes($number);
/**
* Get rateable object score (total)
* @return integer
*/
function getRatingTotal();
/**
* Set rateable object score (total)
* @param integer $number
*/
function setRatingTotal($number);
/**
* Compute the average score of a resource
* @return integer
*/
function getAverageScore();
}
| 34607150811dc34636ecf44c1d3ca34c04a2a9ed | [
"Markdown",
"PHP"
] | 13 | Markdown | squareglasses/DoctrineExtensions-Rateable | 1e3a7c032462160f0c1ee991c0191af422bc34d3 | c77cf90b7e1ae3e28653701edbf479e4426012fd | |
refs/heads/master | <file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using Entidades;
using BLL;
namespace Registros_Tarea_11.Registros
{
public partial class RegistroUsuarios : Form
{
public RegistroUsuarios()
{
InitializeComponent();
}
Utilidades ut = new Utilidades();
private void LlenarClase(Usuarios u)
{
u.Nombre = NombretextBox.Text;
u.Clave = ClavetextBox.Text;
}
private void Guardarbutton_Click(object sender, EventArgs e)
{
Usuarios usuario = new Usuarios();
LlenarClase(usuario);
UsuariosBLL.Insertar(usuario);
MessageBox.Show("Registro Exitoso!!!");
}
private void Buscarbutton_Click(object sender, EventArgs e)
{
BuscarUsuario(UsuariosBLL.Buscar(String(IdtextBox.Text)));
}
public int String(string texto)
{
int numero = 0;
int.TryParse(texto, out numero);
return numero;
}
private void LlenarUsuarios(Usuarios usuario)
{
IdtextBox.Text = usuario.UsuarioId.ToString();
NombretextBox.Text = usuario.Nombre;
ClavetextBox.Text = usuario.Clave;
}
public void BuscarUsuario(Usuarios usuario)
{
if (IdtextBox.Text == "")
{
MessageBox.Show("Para hacer una busqueda de Usuario debe ingresar el ID");
}
else
{
IdtextBox.Text = usuario.UsuarioId.ToString();
NombretextBox.Text = usuario.Nombre.ToString();
ClavetextBox.Text = usuario.Clave.ToString();
}
}
private bool ValidarBuscar()
{
if (UsuariosBLL.Buscar(String(IdtextBox.Text)) == null)
{
MessageBox.Show("Este registro no existe");
return false;
}
return true;
}
private void Nuevobutton_Click(object sender, EventArgs e)
{
IdtextBox.Text = "";
NombretextBox.Text = "";
ClavetextBox.Text = "";
}
private void Eliminarbutton_Click(object sender, EventArgs e)
{
UsuariosBLL.Eliminar(ut.String(IdtextBox.Text));
MessageBox.Show("Eliminado");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using Registros_Tarea_11.Registros;
using Registros_Tarea_11.Consultas;
namespace Registros_Tarea_11
{
public partial class Principal : Form
{
public Principal()
{
InitializeComponent();
}
private void categoriToolStripMenuItem_Click(object sender, EventArgs e)
{
RegistroUsuarios u = new RegistroUsuarios();
u.Show();
}
private void peliculasToolStripMenuItem_Click(object sender, EventArgs e)
{
RegistroPeliculas u = new RegistroPeliculas();
u.Show();
}
private void peliculasToolStripMenuItem1_Click(object sender, EventArgs e)
{
ConsultarUsuario u = new ConsultarUsuario();
u.Show();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.Linq;
using System.Text;
namespace Entidades
{
public class Peliculas
{
[Key]
public int IdPelicula { get; set; }
public DateTime Estreno { get; set; }
public string Descripcion { get; set; }
public int CategoriId { get; set; }
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using Entidades;
using DAL;
namespace BLL
{
public class PeliculasBLL
{
public static void Insertar(Peliculas p)
{
try
{
Registros_tarea11 db = new Registros_tarea11();
db.Pelicula.Add(p);
db.SaveChanges();
db.Dispose();
}
catch (Exception ex)
{
throw ex;
}
}
public static void Eliminar(Peliculas p)
{
Registros_tarea11 db = new Registros_tarea11();
Peliculas peli = db.Pelicula.Find(p);
db.Pelicula.Remove(peli);
db.SaveChanges();
}
public static Peliculas Buscar(int Id)
{
Registros_tarea11 db = new Registros_tarea11();
return db.Pelicula.Find(Id);
}
public static void Eliminar(int v)
{
Registros_tarea11 db = new Registros_tarea11();
Peliculas peli = db.Pelicula.Find(v);
try
{
db.Pelicula.Remove(peli);
db.SaveChanges();
}
catch (Exception ex)
{
throw ex;
}
}
public static List<Peliculas> GetListaPeliculas(string tmp)
{
List<Peliculas> lista = new List<Peliculas>();
Registros_tarea11 db = new Registros_tarea11();
lista = db.Pelicula.Where(p => p.Descripcion == tmp).ToList();
return lista;
}
public static List<Peliculas> GetLista()
{
List<Peliculas> lista = new List<Peliculas>();
Registros_tarea11 db = new Registros_tarea11();
lista = db.Pelicula.ToList();
return lista;
}
public static List<Peliculas> GetLista(int peliculaid)
{
List<Peliculas> lista = new List<Peliculas>();
Registros_tarea11 db = new Registros_tarea11();
lista = db.Pelicula.Where(p => p.IdPelicula == peliculaid).ToList();
return lista;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
using System.Text;
using Entidades;
namespace DAL
{
public class Registros_tarea11:DbContext
{
public Registros_tarea11():base("name = ConStr")
{
}
public virtual DbSet<Usuarios> Usuario { get; set; }
public virtual DbSet<Peliculas> Pelicula { get; set; }
}
}
<file_sep>use Re
create table Usuarios(
UsuarioId int identity (1,1) primary key,
Nombre varchar(20),
Clave varchar(20)
);
create table Peliculas(
IdPelicula int identity (1,1) primary key,
Estreno datetime,
Descripcion varchar(20),
CategoriId int
);
create table Categorias(
CategoriaId int identity (1,1) primary key,
Descripcion varchar(20),
);<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using Entidades;
using BLL;
namespace Registros_Tarea_11.Registros
{
public partial class RegistroPeliculas : Form
{
public RegistroPeliculas()
{
InitializeComponent();
}
Utilidades ut = new Utilidades();
private void LlenarClase(Peliculas p)
{
p.Descripcion = DescripciontextBox.Text;
p.Estreno = EstrenodateTimePicker.Value;
}
private void Guardarbutton_Click(object sender, EventArgs e)
{
Peliculas peli = new Peliculas();
LlenarClase(peli);
//no
PeliculasBLL.Insertar(peli);
MessageBox.Show("Registro Exitoso!!!");
}
private void Buscarbutton_Click(object sender, EventArgs e)
{
//mal
BuscarUsuario(PeliculasBLL.Buscar(String(IdtextBox.Text)));
}
public int String(string texto)
{
int numero = 0;
int.TryParse(texto, out numero);
return numero;
}
private void LlenarPeliculas(Peliculas peli)
{
IdtextBox.Text = peli.IdPelicula.ToString();
DescripciontextBox.Text = peli.Descripcion;
EstrenodateTimePicker.Value = peli.Estreno;
}
public void BuscarUsuario(Peliculas pelicula)
{
if (IdtextBox.Text == "")
{
MessageBox.Show("Para hacer una busqueda de Usuario debe ingresar el ID");
}
else
{
IdtextBox.Text = pelicula.IdPelicula.ToString();
DescripciontextBox.Text = pelicula.Descripcion.ToString();
}
}
private bool ValidarBuscar()
{
if (PeliculasBLL.Buscar(String(IdtextBox.Text)) == null)
{
MessageBox.Show("Este registro no existe");
return false;
}
return true;
}
private void Nuevobutton_Click(object sender, EventArgs e)
{
IdtextBox.Text = "";
DescripciontextBox.Text = "";
}
private void RegistroPeliculas_Load(object sender, EventArgs e)
{
}
}
}
| c4fc73b4fd6e6fea7990cdb7fe538ed40fc7d875 | [
"C#",
"SQL"
] | 7 | C# | edimarcordero0220/Tarea_11_EdimarCM | 7bb6982e8dd0ea3c1381405280fb827ab57ed66e | 83da2b6860fd6f928c01ca992992f05778175fa5 | |
refs/heads/master | <file_sep>using IdentitySample_2.Models;
using System.Web;
using System.Web.Mvc;
using Microsoft.AspNet.Identity.EntityFramework;
using Microsoft.AspNet.Identity;
using System.Threading.Tasks;
using Microsoft.AspNet.Identity.Owin;
using Microsoft.Owin.Security;
namespace IdentitySample_2.Controllers
{
public class AccountController : Controller
{
private IAuthenticationManager AuthenticationManager
{
get
{
return HttpContext.GetOwinContext().Authentication;
}
}
ApplicationDbContext dbContext = new ApplicationDbContext();
// GET: Account/Login
[AllowAnonymous]
public ActionResult Login()
{
return View();
}
[HttpPost]
[AllowAnonymous]
public async Task<ActionResult> Login(LoginViewModel model,string returnUrl)
{
if (ModelState.IsValid)
{
var userStore = new UserStore<ApplicationUser>( dbContext );
var userManager = new UserManager<ApplicationUser>(userStore);
var signInManager = new SignInManager<ApplicationUser, string>(userManager, AuthenticationManager);
var user = new ApplicationUser { UserName = model.UserName };
//sign in the user.
//await signInManager.SignInAsync(user, false, false);
var signInStatus = await signInManager.PasswordSignInAsync(model.UserName, model.Password, false, true);
switch (signInStatus)
{
case SignInStatus.Success:
return RedirectToLocal(returnUrl);
case SignInStatus.LockedOut:
break;
case SignInStatus.RequiresVerification:
break;
case SignInStatus.Failure:
break;
default:
break;
}
}
return View();
}
private ActionResult RedirectToLocal(string returnUrl)
{
if (Url.IsLocalUrl(returnUrl))
{
return Redirect(returnUrl);
}
return RedirectToAction("Home", "Account");
}
public ActionResult SignOut()
{
AuthenticationManager.SignOut(DefaultAuthenticationTypes.ApplicationCookie);
return RedirectToAction("Login", "Account");
}
// GET: Account/Register
[AllowAnonymous]
public ActionResult Register()
{
return View();
}
[HttpPost]
[AllowAnonymous]
public async Task<ActionResult> Register(RegisterViewModel register)
{
if (ModelState.IsValid)
{
var userStore = new UserStore<ApplicationUser>(dbContext);
var userManager = new UserManager<ApplicationUser>(userStore);
var signInManager = new SignInManager<ApplicationUser, string>(userManager, AuthenticationManager);
//find user exixts in db
var user = await userManager.FindAsync(register.UserName, register.Password);
if (user != null)
{
return View();
}
//if not create the user
user = new ApplicationUser { UserName = register.UserName, Email = register.EamilID };
var result = await userManager.CreateAsync(user, register.Password);
if (result.Succeeded)
{
//sign in the user.
//await signInManager.SignInAsync(user, false, false);
var signInStatus = await signInManager.PasswordSignInAsync(register.UserName, register.Password, false, true);
}
return View();
}
else
{
//return with errors
return View();
}
}
[Authorize]
public ActionResult Home()
{
return View();
}
}
} | f8e6b8f682d2267c64799216a6f49d74e7297393 | [
"C#"
] | 1 | C# | SOORAJ-SINGH/customAuthenticationUsingOWIN | 01862316c9e8cd86a9924f6d1ed28f69701cf40a | e4c4605ffb790cd8d0988b11819de2a81113557a | |
refs/heads/master | <file_sep><?php
$log = json_decode($_POST["log"]);
$title = $log ->{'title'};
$description = $log ->{'description'};
$level = $log ->{'level'};
$date = date('Y-m-d H:i:s');
$st = $log ->{'stack_trace'};
$il = $log ->{'inner_log'};
$host_name = "db567447493.db.1and1.com";
$database = "db567447493";
$user_name = "dbo567447493";
$password = "<PASSWORD>";
// Create connection
$conn = new mysqli($host_name, $user_name, $password, $database);
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
if(isset($_POST["log"]))
{
$sql = "INSERT INTO Log(Title, Description, Level, Date, StackTrace, InnerLog)
VALUES ('$title', '$description', $level, '$date', '$st', '$il')";
if ($conn->query($sql) === TRUE) {
echo "New record created successfully";
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
$conn->close();
}
else{
$sql = 'SELECT * FROM Log';
$result = $conn->query($sql);
$list = array();
$i = 0;
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
$list[$i] = $row;
$i++;
}
} else {
echo "0 results";
}
?>
<!doctype html>
<html lang="fr">
<head>
<meta charset="utf-8">
<title>Logs</title>
<link rel="stylesheet" href="http://maxcdn.bootstrapcdn.com/bootstrap/3.2.0/css/bootstrap.min.css">
</head>
<body>
<table class="table table-striped table-hover">
<thead>
<tr>
<td> Date </td>
<td> Title </td>
<td> Description </td>
<td> Level </td>
<td> Stack Trace </td>
<td> Inner Log </td>
</tr>
</thead>
<tbody>
<?php
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
echo '<tr>';
echo '<td>'.$row["Date"].'</td>';
echo '<td>'.$row["Title"].'</td>';
echo '<td>'.$row["Description"].'</td>';
echo '<td>'.$row["Level"].'</td>';
echo '<td>'.$row["StackTrace"].'</td>';
echo '<td>'.$row["InnerLog"].'</td>';
echo '</tr>';
}
} else {
echo "0 results";
}
?>
²</tbody>
</table>
<?php
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
echo $row["Title"];
}
} else {
echo "0 results";
}
?>
</body>
</html>
<?php
$conn->close();
} ?> | 1ad0d899e70bf5f419a21b6554becb1531cf0ed0 | [
"PHP"
] | 1 | PHP | marc68128/PhpLogger | 59ce1435ab48143ff9b20e3501114fbd5470b7dc | 9dbd3ac4e806fded15cd92115bd31d019b38c31b | |
refs/heads/master | <repo_name>alone-zd/flask-blog<file_sep>/README.md
# flask-blog
**演示地址: http://alonezd.top**
博客整体采用flask框架,mysql数据库,进行上线开发。教程会在后面陆续更新
目前已经支持的功能:
1. 个人账户的注册、激活与登录
2. markdown的博文编辑功能及图片上传功能
3. 博文详情页页面的html解析及代码高亮等
4. 文章分页以及分类与分类展示
5. 全局搜索功能
使用:
#### 1、建立mysql数据库,我的库名为(alone_blog),或者改为你们自己的库,并在项目目录下的App/config.py里面进行更改。
#### 2、删除migrations文件,然后依次执行
- python3 manage.py db init
- python3 manage.py db migrate
- python3 manage.py db upgrade
#### 3、执行命令行进行启动
```python
python3 manage.py runserver
```
这个时候打开你的本地浏览器,输入 http://127.0.0.1:5000 就可以看到博客页面啦
<file_sep>/App/views/__init__.py
from .main import main # 导入main蓝本对象
from .user import user # 导入user蓝本对象
from .posts import posts # 导入posts博客处理的蓝本对象
from .owncenter import owncenter # 导入owncenter个人中心蓝本对象
# 循环迭代的蓝本配置
blueprint_config = [
(main, ''),
(user, ''),
(posts, ''),
(owncenter, ''),
]
# 注册蓝本对象的函数
def register_blueprint(app):
# 循环迭代注册蓝本
for blueprint,perfix in blueprint_config:
app.register_blueprint(blueprint,url_prefix=perfix)<file_sep>/App/forms/__init__.py
from .user import Register,Login,AgainActive
from .posts import SendPosts,Comment,Catgs
from .owncenter import UserInfo,Upload<file_sep>/App/views/posts.py
from flask import Blueprint, render_template, flash, redirect, url_for, current_app
from sqlalchemy import or_
from App.forms import SendPosts, Comment, Catgs # 导入发表博客与发表评论的表单类
from App.models import Posts,Categorys,User # 导入博客模型类和标签模型类
from flask_login import current_user, login_required
from datetime import datetime
from flask import jsonify, request
from App.extensions import qiniu_store, csrf
import os, base64
from App.extensions import file
from PIL import Image
posts = Blueprint('posts', __name__)
# 使用uuid扩展库生成唯一的名称
def random_filename(suffix):
import uuid
u = uuid.uuid4()
return str(u) + '.' + suffix
# 图片缩放处理
def img_zoom(path, width=1000, height=250):
# 打开文件
img = Image.open(path)
# 重新设计尺寸
# img.thumbnail((width, height))
# 保存缩放后的图片 保留原图片
# 保存缩放
img.save(path)
@posts.route('/send_posts/', methods=['GET', 'POST'])
def send_posts():
form = SendPosts()
ctgs = Categorys.query.all()
# 判断登录后在进行发表
if not current_user.is_authenticated:
flash('您还没有登录 请登录后再发表')
if current_user.id==1:
if form.validate_on_submit():
# md格式
article = request.form['article']
ctgs = request.form['ctgs']
tags = Categorys.query.filter_by(categorys=ctgs).first()
# 图片上传
img = request.files.get('img') # 获取上传对象
ex = os.path.splitext(img.filename)[1]
filename = datetime.now().strftime('%Y%m%d%H%M%S') + ex
file = img.stream.read()
qiniu_store.save(file, filename)
post = Posts(title=form.title.data, user=current_user, article=article, img=qiniu_store.url(filename),tags=[tags])
post.save()
flash('博客发表成功')
return redirect(url_for('main.index'))
else:
flash('您没有发表博客的权限!')
return redirect(url_for('main.index'))
return render_template('posts/send_posts.html', form=form, ctgs=ctgs)
# 增加分类
@posts.route('/add_catgs/', methods=['GET', 'POST'])
def add_catgs():
form = Catgs()
ctgs = Categorys.query.all()
if not current_user.is_authenticated:
flash('您还没有登录 请登录后再操作')
elif form.validate_on_submit():
catgs = Categorys(categorys=form.categorys.data)
catgs.save()
print(form.categorys.data)
flash('分类添加成功')
return redirect(url_for('posts.send_posts'))
return render_template('posts/add_categorys.html', form=form, ctgs=ctgs)
# 删除分类
@posts.route('/delete_catgs/', methods=['GET', 'POST'])
def delete_catgs():
ctgs = Categorys.query.all()
form = Catgs()
if not current_user.is_authenticated:
flash('您还没有登录 请登录后再操作')
elif form.validate_on_submit():
cs = request.form['ctgs']
d = Categorys.query.filter_by(categorys=cs)[0]
d.delete()
flash('分类删除成功')
return redirect(url_for('posts.send_posts'))
return render_template('posts/delete_categorys.html', form=form, ctgs=ctgs)
# 搜索
@posts.route('/search/', methods=['GET', 'POST'])
@csrf.exempt
def search():
try:
page = int(request.args.get('page'))
except:
page = 1
# 获取搜索的关键字
if request.method == 'POST':
keyword = request.form.get('keyword', '')
else:
keyword = request.args.get('keyword', '')
# 查询pid为0(是博客内容,不为0则是评论或回复内容),statue为0(所有人可见),按照时间降序显示
# 搜索功能,搜索内容为:标题或正文
pagination = Posts.query.filter(or_(Posts.title.contains(keyword), Posts.article.contains(keyword)), Posts.pid == 0,
Posts.state == 0) \
.order_by(Posts.timestamp.desc()) \
.paginate(page, current_app.config['PAGE_NUM'], False) # False 为不抛出异常
data = pagination.items # 获取page页面的数据
# 将文章(pid=0)对应的的id查询出来,
art = Posts.query.filter(Posts.pid==0).count()
# 按照访问量排行降序查询,拿出前五
visit = Posts.query.filter(Posts.pid == 0, Posts.state == 0).order_by(-Posts.visit)[:5]
# 拿到文章对象,进行遍历,然后将访问量放进数组,进行求和
article_list = Posts.query.filter(Posts.pid==0)
list = []
for i in article_list:
list.append(i.visit)
v=sum(list)
cs = Categorys.query.all()
comments_five = Posts.query.filter(Posts.pid!=0)[0:5]
return render_template('posts/search_detail.html', pagination=pagination, posts=data, keyword=keyword,art = art, visit=visit, v=v, ctgs=cs,comments_five=comments_five)
# 博客分类
@posts.route('posts_ctgs',methods=['GET','POST'])
def posts_ctgs():
try:
page = int(request.args.get('page'))
except:
page = 1
cid = request.args.get('pid')
global pagination,data
posts = Categorys.query.get(cid)
for i in posts.posts:
pagination = Posts.query.filter(Posts.title==i.title).order_by(Posts.timestamp.desc()).paginate(page, current_app.config['PAGE_NUM'], False) # False 为不抛出异常
data = pagination.items # 获取page页面的数据
category = Categorys.query.get(cid)
# 将文章(pid=0)对应的的id查询出来,
art = Posts.query.filter(Posts.pid==0).count()
# 按照访问量排行降序查询,拿出前五
visit = Posts.query.filter(Posts.pid == 0, Posts.state == 0).order_by(-Posts.visit)[:5]
# 拿到文章对象,进行遍历,然后将访问量放进数组,进行求和
article_list = Posts.query.filter(Posts.pid==0)
list = []
for i in article_list:
list.append(i.visit)
v=sum(list)
cs = Categorys.query.all()
comments_five = Posts.query.filter(Posts.pid!=0)[0:5]
return render_template('posts/posts_ctgs.html',pagination=pagination,posts=data,category=category,art = art, visit=visit, v=v, ctgs=cs,comments_five=comments_five)
# 博客详情
@posts.route('/posts_detail/<int:pid>/')
def posts_detail(pid):
# 查询当前博客的内容 pid为博客的自增id 并不是博客模型字段的pid
p = Posts.query.get(pid)
p.visit += 1 # 博客的访问量
p.save()
form = Comment() # 发表评论和回复的表单类
# 查询出博客的评论内容和回复,按照博客内容和回复在一起的排序顺序,
# 过滤条件为博客path中包含博客id的,这样博客就将博客的内容过滤出去,只查询评论和留言
comment = Posts.query.filter(Posts.path.contains(str(pid))).order_by(Posts.path.concat(Posts.id))
ctgs = Categorys.query.all()
comment_nums = Posts.query.filter(Posts.path.contains(str(pid))).count()
return render_template('posts/posts_detail.html', posts=p, form=form, comment=comment, ctgs=ctgs, comment_nums=comment_nums)
# 评论和回复
@posts.route('/comment/', methods=['GET', 'POST'])
@login_required
def comment():
pid = request.form.get('pid')
rid = request.form.get('rid')
# 判断当前是评论还是回复,如果为评论则为假,否则为真
if rid:
id = rid
else:
id = pid
p = Posts.query.get(int(id))
Posts(article=request.form.get('article'), pid=id, path=p.path + id + ',', user=current_user).save()
return redirect(url_for('posts.posts_detail', pid=pid))
# editor.md的图片上传
@posts.route('/upload_image/', methods=['POST'])
@login_required
@csrf.exempt
def upload_image():
data = request.files.get('editormd-image-file')
if not data:
res = {
'success': 0,
'message': '图片失败请重试'
}
else:
ex = os.path.splitext(data.filename)[1]
filename = datetime.now().strftime('%Y%m%d%H%M%S') + ex
file = data.stream.read()
qiniu_store.save(file, filename)
res = {
'success': 1,
'message': '图片上传成功',
'url': qiniu_store.url(filename)
}
return jsonify(res)
<file_sep>/App/__init__.py
from flask import Flask
from App.views import register_blueprint # 导入views注册蓝本对象的方法
from .config import configDict # 导入配置类的字典
from .extensions import init_app # 导入初始化扩展库的init_app方法
from App.models import Posts
# 加载整个App项目并返回App对象
def create_app(configName='default'):
app = Flask(__name__)
# 加载配置类
app.config.from_object(configDict[configName])
from datetime import timedelta
app.config['SEND_FILE_MAX_AGE_DEFAULT'] = timedelta(seconds=1)
# 注册蓝本
register_blueprint(app)
# 初始化扩展库
init_app(app)
# 添加自定义模板过滤器
addTemFilter(app)
return app
# 自定义模板过滤器
def addTemFilter(app):
# 内容超出给定的长度 显示...
def showEllipsis(Str, length=150):
if len(Str)>length:
Str = Str[0:length]+' · · · · · · · · '
return Str
# 获取回复人的名称的过滤器
def replayName(pid):
username = Posts.query.get(int(pid)).user.username
return username
app.add_template_filter(showEllipsis)
app.add_template_filter(replayName)
<file_sep>/App/models/user.py
from App.extensions import db # 导入模型对象
from .db_base import DB_Base # 导入自定义模型基类
from datetime import datetime
# 导入生成与验证密码的方法
from werkzeug.security import generate_password_hash, check_password_hash
from itsdangerous import TimedJSONWebSignatureSerializer as Seralize # 导入序列化方法
from flask import current_app
from flask_login import UserMixin # 包含了判断是否登录等等方法饿基类
from App.extensions import login_manager
# User 模型类
class User(UserMixin,db.Model, DB_Base):
__tablename__ = 'user'
id = db.Column(db.Integer, primary_key=True) # 自增id
username = db.Column(db.String(12), index=True, unique=True) # 用户名
password_hash = db.Column(db.String(128)) # 加密的密码
sex = db.Column(db.Boolean, default=True) # 性别
age = db.Column(db.Integer, default=18) # 年龄
email = db.Column(db.String(50), unique=True) # 邮箱
icon = db.Column(db.String(70), default='default.jpg') # 头像
lastLogin = db.Column(db.DateTime) # 上次登录时间
registerTime = db.Column(db.DateTime, default=datetime.utcnow) # 注册时间
confirm = db.Column(db.Boolean, default=False) # 激活状态,默认未激活
# 设置模型引用关系
"""
Posts : 建立引用关系的模型
backref : 是给关联的模型添加一个查询的属性 叫user
lazy : 加载的时机 返回查询集(可以添加连贯操作)
"""
posts = db.relationship('Posts',backref='user',lazy='dynamic')
# 密码加密处理的装饰器
@property
def password(self):
raise AttributeError
@password.setter
def password(self, password):
self.password_hash = <PASSWORD>_password_hash(password)
# 检验密码正确性的方法
def check_password(self, password):
return check_password_hash(self.password_hash, password)
# 生成token用于账户激活(也就是知道事哪个用户激活的)
def generate_token(self):
s = Seralize(current_app.config['SECRET_KEY'])
return s.dumps({'id': self.id}) # 返回加密的字符串
# 检测传递过来的token值, 进行账户激活
@staticmethod
def check_token(token):
s = Seralize(current_app.config['SECRET_KEY'])
try:
id = s.loads(token)['id']
u = User.query.get(id)
if not u:
return False
u.confirm = True
u.save()
return True
except:
return False
# 这是一个回调的方法,实时获取当前表中用户数据的对象
@login_manager.user_loader
def user_loader(userid):
return User.query.get(int(userid))
<file_sep>/App/models/posts.py
from App.extensions import db
from .db_base import DB_Base
from datetime import datetime
posts_categorys = db.Table('posts_categorys',
db.Column('posts_id', db.Integer, db.ForeignKey('posts.id'), primary_key=True),
db.Column('categorys_id', db.Integer, db.ForeignKey('categorys.id'), primary_key=True)
)
# 博客模型
class Posts(DB_Base, db.Model):
__tablename__ = 'posts'
id = db.Column(db.Integer, primary_key=True)
title = db.Column(db.String(20), index=True) # 标题
article = db.Column(db.Text) # md格式正文
pid = db.Column(db.Integer, default=0) # 父id
path = db.Column(db.Text, default='0,') # 路径
visit = db.Column(db.Integer, default=0) # 文章访问量
timestamp = db.Column(db.DateTime, default=datetime.utcnow) # 发表时间
state = db.Column(db.Integer, default=0) # 是否所有人可见
img = db.Column(db.String(70)) # 图片
tags = db.relationship('Categorys', secondary=posts_categorys, backref=db.backref('posts'))
u_id = db.Column(db.Integer, db.ForeignKey('user.id')) # 关联主表user的自增id
class Categorys(DB_Base, db.Model):
__tablename__ = 'categorys'
id = db.Column(db.Integer, primary_key=True)
categorys = db.Column(db.String(20),index=True, unique=True)
<file_sep>/migrations/versions/f9c3ac552084_.py
"""empty message
Revision ID: f9c3ac552084
Revises: <PASSWORD>
Create Date: 2020-04-08 16:54:02.195068
"""
from alembic import op
import sqlalchemy as sa
from sqlalchemy.dialects import mysql
# revision identifiers, used by Alembic.
revision = 'f9c3ac552084'
down_revision = '<PASSWORD>'
branch_labels = None
depends_on = None
def upgrade():
# ### commands auto generated by Alembic - please adjust! ###
op.drop_constraint('posts_ibfk_2', 'posts', type_='foreignkey')
op.drop_column('posts', 'c_id')
# ### end Alembic commands ###
def downgrade():
# ### commands auto generated by Alembic - please adjust! ###
op.add_column('posts', sa.Column('c_id', mysql.INTEGER(display_width=11), autoincrement=False, nullable=True))
op.create_foreign_key('posts_ibfk_2', 'posts', 'categorys', ['c_id'], ['id'])
# ### end Alembic commands ###
<file_sep>/App/forms/user.py
from flask_wtf import FlaskForm
from wtforms import StringField, PasswordField, SubmitField, BooleanField
from wtforms.validators import DataRequired, Length, EqualTo, Email, ValidationError
from App.models import User # 导入User模型类
# 注册表单类
class Register(FlaskForm):
username = StringField('用户名', validators=[DataRequired('用户名不能为空'), Length(min=4, max=12, message='用户名在4-12位之间')],
render_kw={'placeholder': '请输入用户名...'})
userpass = PasswordField('密码', validators=[DataRequired('密码不能为空')], render_kw={'placeholder': '请输入密码...'})
confirm = PasswordField('确认密码', validators=[DataRequired('确认密码不能为空...'), EqualTo('userpass', message='密码和确认密码不一致')],
render_kw={'placeholder': '请输入确认密码...'})
email = StringField('激活邮箱', validators=[DataRequired('邮箱地址不能为空'), Email(message='请输入正确的邮箱地址')],
render_kw={'placeholder': '请输入用于账户激活的邮箱地址...'})
submit = SubmitField('注册')
# 验证用户名是否存在
def validate_username(self, field):
if User.query.filter_by(username=field.data).first():
raise ValidationError('该用户名已存在,请重新输入')
# 验证邮箱是否存在
def validate_email(self, field):
if User.query.filter_by(email=field.data).first():
raise ValidationError('该邮箱已存在,请重新输入')
# 登录表单类
class Login(FlaskForm):
username = StringField('用户名', validators=[DataRequired('用户名不能为空'), Length(min=4, max=12, message='用户名在4-12位之间')],
render_kw={'placeholder': '请输入用户名...'})
userpass = PasswordField('密码', validators=[DataRequired('密码不能为空')], render_kw={'placeholder': '请输入密码...'})
remember = BooleanField('记住我', default='checked')
submit = SubmitField('登录')
# 验证用户名是否存在
def validate_username(self, field):
if not User.query.filter_by(username=field.data).first():
raise ValidationError('请输入正确的用户名或密码')
# 再次激活表单类
class AgainActive(FlaskForm):
username = StringField('用户名', validators=[DataRequired('用户名不能为空'), Length(min=4, max=12, message='用户名在4-12位之间')],
render_kw={'placeholder': '请输入用户名...'})
userpass = PasswordField('密码', validators=[DataRequired('密码不能为空')], render_kw={'placeholder': '请输入密码...'})
submit = SubmitField('激活')
# 验证用户名是否存在
def validate_username(self, field):
if not User.query.filter_by(username=field.data).first():
raise ValidationError('请输入正确的用户名或密码')<file_sep>/requirements.txt
alembic==1.3.3
bleach==3.1.2
blinker==1.4
certifi==2019.11.28
chardet==3.0.4
Click==7.0
dominate==2.4.0
Flask==1.1.1
Flask-Bootstrap==3.3.7.1
Flask-Login==0.4.1
Flask-Mail==0.9.1
Flask-Markdown==0.3
flask-markdown2==0.0.3
Flask-Migrate==2.5.2
Flask-Moment==0.9.0
Flask-QiniuStorage==0.9.5
Flask-Script==2.0.6
Flask-SQLAlchemy==2.4.1
Flask-Uploads==0.2.1
Flask-WTF==0.14.2
idna==2.9
itsdangerous==1.1.0
Jinja2==2.11.1
jinja2-markdown==0.0.3
Mako==1.1.1
Markdown==3.2.1
MarkupSafe==1.1.1
mysql-connector==2.2.9
Pillow==7.0.0
PyMySQL==0.9.3
python-dateutil==2.8.1
python-editor==1.0.4
qiniu==7.2.6
requests==2.23.0
six==1.14.0
SQLAlchemy==1.3.13
urllib3==1.25.8
visitor==0.1.3
webencodings==0.5.1
Werkzeug==0.16.1
WTForms==2.2.1
<file_sep>/migrations/versions/0403e1b46505_.py
"""empty message
Revision ID: 0403e1b46505
Revises: <PASSWORD>
Create Date: 2020-04-07 22:32:53.535757
"""
from alembic import op
import sqlalchemy as sa
from sqlalchemy.dialects import mysql
# revision identifiers, used by Alembic.
revision = '<KEY>'
down_revision = '<PASSWORD>'
branch_labels = None
depends_on = None
def upgrade():
# ### commands auto generated by Alembic - please adjust! ###
op.create_table('posts_categorys',
sa.Column('posts_id', sa.Integer(), nullable=False),
sa.Column('categorys_id', sa.Integer(), nullable=False),
sa.ForeignKeyConstraint(['categorys_id'], ['categorys.id'], ),
sa.ForeignKeyConstraint(['posts_id'], ['posts.id'], ),
sa.PrimaryKeyConstraint('posts_id', 'categorys_id')
)
op.drop_constraint('categorys_ibfk_1', 'categorys', type_='foreignkey')
op.drop_column('categorys', 'aid')
op.add_column('posts', sa.Column('u_id', sa.Integer(), nullable=True))
op.drop_constraint('posts_ibfk_2', 'posts', type_='foreignkey')
op.create_foreign_key(None, 'posts', 'user', ['u_id'], ['id'])
op.drop_column('posts', 'uid')
# ### end Alembic commands ###
def downgrade():
# ### commands auto generated by Alembic - please adjust! ###
op.add_column('posts', sa.Column('uid', mysql.INTEGER(display_width=11), autoincrement=False, nullable=True))
op.drop_constraint(None, 'posts', type_='foreignkey')
op.create_foreign_key('posts_ibfk_2', 'posts', 'user', ['uid'], ['id'])
op.drop_column('posts', 'u_id')
op.add_column('categorys', sa.Column('aid', mysql.INTEGER(display_width=11), autoincrement=False, nullable=True))
op.create_foreign_key('categorys_ibfk_1', 'categorys', 'posts', ['aid'], ['id'])
op.drop_table('posts_categorys')
# ### end Alembic commands ###
<file_sep>/App/extensions.py
from flask_bootstrap import Bootstrap
from flask_sqlalchemy import SQLAlchemy # ORM模型扩展库
from flask_migrate import Migrate # 模型迁移
from flask_mail import Mail # 邮件发送扩展库
from flask_login import LoginManager # 处理用户登录的扩展库
from flask_moment import Moment # 格式化时间显示的扩展库
from flask_uploads import IMAGES, UploadSet,configure_uploads,patch_request_class # 导入头像上传
from flask_qiniustorage import Qiniu # 七牛云上传
from flask_wtf.csrf import CSRFProtect
# 实例化
bootstrap = Bootstrap() # bootstrap扩展库
db = SQLAlchemy() # ORM模型
migrate = Migrate() # 模型迁移
mail = Mail() # 邮件发送
login_manager = LoginManager() # 处理登录的库
moment = Moment() # 格式化时间显示的扩展库
file = UploadSet('photos',IMAGES) # 上传头像的
qiniu_store = Qiniu()
csrf = CSRFProtect()
# 加载app
def init_app(app):
bootstrap.init_app(app)
db.init_app(app)
migrate.init_app(app=app, db=db)
mail.init_app(app)
moment.init_app(app)
login_manager.init_app(app)
qiniu_store.init_app(app)
csrf.init_app(app)
login_manager.login_view = 'user.login' # 当未登陆时,访问了需要登录的路由时,进行登录的端点
login_manager.session_protection = 'basic' # 设置session的保护级别
# 配置文件上传(头像)
configure_uploads(app,file)
patch_request_class(app, size=None)
<file_sep>/App/forms/posts.py
from flask_wtf import FlaskForm
from wtforms import StringField, TextAreaField, SubmitField
from wtforms.validators import DataRequired, Length
from flask_wtf.file import FileAllowed, FileRequired, FileField
from App.extensions import file # 导入文件上传配置对象
# 发表博客的表单类
class SendPosts(FlaskForm):
title = StringField('标题', validators=[DataRequired('标题内容不能为空'),
Length(min=3,max=100,message='标题内容在3-100字之间')],render_kw={'placeholder':'请输入标题'})
article = TextAreaField('博客内容', validators=[DataRequired('博客内容不能为空')],render_kw={'placeholder':'请输入博客内容'})
submit = SubmitField('发表博客')
# 发表评论和回复的表单类
class Comment(FlaskForm):
article = TextAreaField('评论内容', validators=[DataRequired('评论内容不能为空')])
submit = SubmitField('发表评论')
# 添加分类的表单
class Catgs(FlaskForm):
categorys = StringField('分类专栏',
render_kw={'placeholder': '请输入分类'})
<file_sep>/manage.py
from flask import Flask
from flask_script import Manager
# 导入创建App,并加载整个项目的方法
from App import create_app
from flask_migrate import MigrateCommand
# 调用
app = create_app()
manager = Manager(app)
manager.add_command('db', MigrateCommand)
if __name__ == '__main__':
manager.run()<file_sep>/App/views/user.py
from flask import Blueprint,render_template,flash,url_for,redirect
from App.forms import Register, Login, AgainActive # 导入表单注册类
from App.models import User # 导入User模型类
from App.email import send_mail # 导入发送邮件的函数
from flask_login import login_required, logout_user, login_user, current_user
from datetime import datetime
user = Blueprint('user', __name__)
@user.route('/register/', methods=['GET', 'POST'])
def register():
# 实例化注册表单类
form = Register()
if form.validate_on_submit():
# 实例化存储注册表单数据
u = User(username=form.username.data, password=<PASSWORD>,email=form.email.data)
u.save()
# 调用获取token的方法
token = u.generate_token()
# 发送邮件激活
send_mail('账户激活', form.email.data,username=form.username.data, token=token)
flash('注册成功,请前去邮箱激活')
# 成功去登录
return redirect(url_for('user.login'))
return render_template('user/register.html', form=form)
# 进行账户激活的视图
@user.route('/active/<token>')
def active(token):
if User.check_token(token):
flash('激活成功,请前去登录...')
# 激活成功跳转到登录
return redirect(url_for('user.login'))
else:
flash('激活失败,请重新进行账户激活操作....')
return redirect(url_for('user.again_active'))
# 再次激活的视图
@user.route('/again_active/',methods=['GET', 'POST'])
def again_active():
form = AgainActive()
if form.is_submitted():
u = User.query.filter(User.username == form.username.data).first()
if not u:
flash('请输入正确的用户名或密码....')
elif not u.check_password(form.userpass.data):
flash('请输入正确的用户名或密码')
elif not u.confirm:
# 调用获取token的方法
token = u.generate_token()
# 发送邮件激活
send_mail('账户再次激活', u.email,tem='again_active', username=form.username.data, token=token)
flash('激活邮件发送成功,请前去邮箱激活')
else:
flash('该账户已经激活,请前去登录...')
return render_template('user/again_active.html', form=form)
# 登录视图
@user.route('/login/', methods=['GET', 'POST'])
def login():
form = Login()
if form.validate_on_submit():
u = User.query.filter(User.username == form.username.data).first()
if not u:
flash('请输入正确的用户名或密码')
elif not u.confirm:
flash('您还没有进行激活,请前去激活账户')
return redirect(url_for('user.again_active'))
elif not u.check_password(form.userpass.data):
flash('请输入正确的用户名或密码')
else:
flash('登录成功')
# 修改登录时间
u.lastLogin = datetime.now()
u.save()
login_user(u, remember=form.remember.data) # 使用第三方扩展库处理登录状态的维持
return redirect(url_for('main.index'))
return render_template('user/login.html', form=form)
# 退出登录
@user.route('logout')
def logout():
logout_user()
flash('退出成功')
return redirect(url_for('main.index'))<file_sep>/App/models/db_base.py
from App.extensions import db
# 自定义封装模型基类
class DB_Base:
# 添加一条数据
def save(self):
try:
# self 代表当前当前实例化的对象
db.session.add(self)
db.session.commit()
return True
except:
db.session.rollback()
return False
# 添加多条数据
@staticmethod
def save_all(*args):
try:
db.session.add_all(args)
db.session.commit()
return True
except:
db.session.rollback()
return False
# 删除
def delete(self):
try:
db.session.delete(self)
db.session.commit()
return True
except:
db.session.rollback()
return False<file_sep>/App/views/main.py
from flask import Blueprint,render_template, request,current_app
from App.models import Posts,Categorys
from sqlalchemy import func
main = Blueprint('main', __name__)
# 首页视图
@main.route('/')
@main.route('/index/')
def index():
try:
page = int(request.args.get('page',1))
except:
page = 1
# 查询pid为0(是博客内容,不为0则是评论或回复内容),statue为0(所有人可见),按照时间降序显示
pagination = Posts.query.filter(Posts.pid==0, Posts.state==0).order_by(Posts.timestamp.desc())\
.paginate(page, current_app.config['PAGE_NUM'], False) # False 为不抛出异常
data = pagination.items # 获取page页面的数据
# 将文章(pid=0)对应的的id查询出来,
art = Posts.query.filter(Posts.pid==0).count()
# 按照访问量排行降序查询,拿出前五
visit = Posts.query.filter(Posts.pid == 0, Posts.state == 0).order_by(-Posts.visit)[:5]
# 拿到文章对象,进行遍历,然后将访问量放进数组,进行求和
article_list = Posts.query.filter(Posts.pid==0)
list = []
for i in article_list:
list.append(i.visit)
v=sum(list)
cs = Categorys.query.all()
comments_five = Posts.query.filter(Posts.pid!=0)[0:5]
return render_template('main/index.html', posts=data, pagination=pagination, art = art, visit=visit, v=v, ctgs=cs,comments_five=comments_five)
<file_sep>/uwsgi.ini
[uwsgi]
socket = 0.0.0.0:8000
chdir = /home/flask-blog/
wsgi-file = manage.py
callable =app
processes = 1
threads = 2
<file_sep>/App/config.py
import os
from datetime import timedelta
BASE_DIR = os.path.abspath(os.path.dirname(__file__))
# 配置类的基类
class Config:
SECRET_KEY = 'alone'
SQLALCHEMY_TRACK_MODIFICATIONS = False
BOOTSTRAP_SERVER_LOCAL = True # 加载本地静态资源文件
# 邮件发送配置
# 端口号
MAIL_PORT = 465
MAIL_USE_SSL = True
# 邮箱服务器
MAIL_SERVER = 'smtp.163.com'
# 用户名
MAIL_USERNAME = '<EMAIL>'
# 密码
MAIL_PASSWORD = '<PASSWORD>'
# 分页每页显示数据条数
PAGE_NUM = 6
# 文件上传配置
UPLOADED_PHOTOS_DEST = os.path.join(BASE_DIR, 'static/upload')
MAX_CONTENT_LENGTH = 1024 * 1024 * 64
# 七牛云上传配置
QINIU_ACCESS_KEY = "<KEY>"
QINIU_SECRET_KEY = "<KEY>"
QINIU_BUCKET_NAME = "aloneblog"
QINIU_BUCKET_DOMAIN = 'cdnonline.top'
# 开发环境
class DevelopmentConfig(Config):
SQLALCHEMY_DATABASE_URI = 'mysql+mysqlconnector://root:[email protected]:3306/alone_blog'
DEBUG = True
TESTING = False
# 测试环境
class TestingConfig(Config):
SQLALCHEMY_DATABASE_URI = 'mysql+mysqlconnector://root:[email protected]:3306/test_blog'
DEBUG = False
TESTING = True
# 生产环境
class ProductionConfig(Config):
SQLALCHEMY_DATABASE_URI = 'mysql+mysqlconnector://root:[email protected]:3306/blog'
DEBUG = False
TESTING = False
# 配置类的字典,给类起别名
configDict = {
'default': DevelopmentConfig,
'dev': DevelopmentConfig,
'Test': TestingConfig,
'production': ProductionConfig,
}
<file_sep>/App/forms/owncenter.py
from flask_wtf import FlaskForm
from wtforms import StringField, SubmitField, IntegerField, RadioField
from wtforms.validators import DataRequired, NumberRange
from flask_wtf.file import FileAllowed, FileRequired, FileField
from App.extensions import file # 导入文件上传配置对象
# 修改与查看个人信息的表单类
class UserInfo(FlaskForm):
username = StringField('用户名', render_kw={'readonly': 'true'})
sex = RadioField(label='性别', choices=[('1', '男'), ('0', '女')], validators=[DataRequired('性别必选')])
age = IntegerField('年龄', validators=[DataRequired('年龄不能为空'), NumberRange(min=1, max=99, message='年龄在1~99之间')])
email = StringField('邮箱', render_kw={'readonly': 'true'})
lastLogin = StringField('上次登录时间', render_kw={'disabled': 'true'})
register = StringField('注册时间', render_kw={'disabled': 'true'})
submit = SubmitField('修改')
# 文件上传
class Upload(FlaskForm):
icon = FileField('头像上传', validators=[FileRequired('您还没有选择上传的头像'),FileAllowed(file, message='该文件类型不允许上传')])
submit = SubmitField('上传')<file_sep>/App/views/owncenter.py
from datetime import datetime
from flask import Blueprint, render_template, redirect, url_for, flash, request, current_app
from App.models import Posts, Categorys
from App.forms import UserInfo # 导入个人信息显示user模型类和文件上传表单类
from App.forms import SendPosts,Upload # 用于编辑博客
from flask_login import current_user, login_required
from App.extensions import db, file, qiniu_store
import os
from App.extensions import file
from PIL import Image
owncenter = Blueprint('owncenter', __name__)
# 查看与修改个人信息
@owncenter.route('/user_info/', methods=['GET', 'POST'])
def user_info():
form = UserInfo()
if form.validate_on_submit():
current_user.age = form.age.data
form.username.data = current_user.username
form.age.data = current_user.age
form.sex.data = str(int(current_user.sex))
form.email.data = current_user.email
form.lastLogin.data = current_user.lastLogin
form.register.data = current_user.registerTime
return render_template('owncenter/user_info.html', form=form)
# 博客管理
@owncenter.route('/posts_manager/')
def posts_manager():
try:
page = int(request.args.get('page', 1))
except:
page = 1
# 查询当前用户发表的所有博客,pid为0证明是博客内容,而不是评论和回复内容。state=0是所有人可见,
# 按照时间进行降序查询
posts = current_user.posts.filter_by(pid=0, state=0).order_by(Posts.timestamp.desc())
# 获取page页面的数据
pagination = Posts.query.filter(Posts.pid == 0, Posts.state == 0).order_by(Posts.timestamp.desc()) \
.paginate(page, current_app.config['PAGE_NUM'], False) # False 为不抛出异常
data = pagination.items # 获取page页面的数据
return render_template('owncenter/posts_manager.html', posts=posts, pagination=pagination)
# 博客删除
@owncenter.route('/del_posts/<int:pid>')
@login_required
def del_posts(pid):
# 查询博客
p = Posts.query.get(pid)
# 判断该博客是否存在
if p:
# 执行删除
flash('删除成功')
p.delete() # 删除博客内容
comment = Posts.query.filter(Posts.path.contains(str(pid))) # 删除评论和留言的内容
for posts in comment:
posts.delete()
else:
flash('您要删除的博客不存在')
return redirect(url_for('owncenter.posts_manager'))
# 使用uuid扩展库生成唯一的名称
def random_filename(suffix):
import uuid
u = uuid.uuid4()
return str(u) + '.' + suffix
# 图片缩放处理
def img_zoom(path, width=100, height=100):
# 打开文件
img = Image.open(path)
# 重新设计尺寸
img.thumbnail((width, height))
# 保存缩放后的图片 保留原图片
# 保存缩放
img.save(path)
# 头像上传
@owncenter.route('/upload/', methods=['GET', 'POST'])
@login_required
def upload():
form = Upload()
if form.validate_on_submit():
icon = request.files.get('icon') # 获取上传对象
suffix = icon.filename.split('.')[-1] # 获取后缀
newName = random_filename(suffix) # 获取新图片的名称
# 以新名称保存图片
file.save(icon, name=newName)
delPath = current_app.config['UPLOADED_PHOTOS_DEST']
# 删除之前上传的图片
if current_user.icon != 'default.jpg': # 如果不等于,则证明上传了头像
os.remove(os.path.join(delPath, current_user.icon))
current_user.icon = newName # 更改当前对象的图片名称
db.session.add(current_user) # 更新到数据库中
db.session.commit()
# 拼接图片路径
path = os.path.join(delPath, newName)
# 进行缩放
img_zoom(path)
return render_template('owncenter/upload.html', form=form)
# 博客编辑
@owncenter.route('/edit_posts/<int:pid>', methods=['POST', 'GET'])
def edit_posts(pid):
form = SendPosts() # 实例化表单
p = Posts.query.get(pid) # 根据博客id 查询
ctgs = Categorys.query.all()
if not p:
flash('该博客不存在')
return redirect(url_for('owncenter.posts_manager'))
if form.validate_on_submit():
# md格式
article = request.form['article']
# 得到所选的分类值
ctgs = request.form['ctgs']
tags = Categorys.query.filter_by(categorys=ctgs).first()
# 图片上传
img = request.files.get('img') # 获取上传对象
ex = os.path.splitext(img.filename)[1]
filename = datetime.now().strftime('%Y%m%d%H%M%S') + ex
file = img.stream.read()
qiniu_store.save(file, filename)
p.title = form.title.data
p.article = article
p.img = qiniu_store.url(filename)
p.tags = [tags]
p.save()
flash('博客更新成功')
return redirect(url_for('owncenter.posts_manager'))
form.title.data = p.title
form.article.data = p.article
return render_template('owncenter/edit_posts.html', form=form, ctgs=ctgs)
<file_sep>/App/models/__init__.py
from .user import User
from .posts import Posts,Categorys
<file_sep>/App/email.py
from flask import render_template, current_app
# 导入线程类
from threading import Thread
from flask_mail import Message
from App.extensions import mail
# 异步执行耗时操作
def async_send_mail(app, msg):
with app.app_context():
mail.send(message=msg)
def send_mail(subject, to, tem='active', **kwargs):
app = current_app._get_current_object()
# 创建邮件对象
msg = Message(subject=subject, recipients=[to], sender=app.config['MAIL_USERNAME'])
# 邮件主体内容
msg.html = render_template('email/' + tem + '.html', **kwargs)
# 发送邮件
# 创建线程
thr = Thread(target=async_send_mail, args=(app, msg))
# 启动线程
thr.start()
return '发送邮件'<file_sep>/migrations/versions/1ed826de7a78_.py
"""empty message
Revision ID: 1<PASSWORD>
Revises: 685d91fb9b42
Create Date: 2020-04-07 21:03:54.087500
"""
from alembic import op
import sqlalchemy as sa
from sqlalchemy.dialects import mysql
# revision identifiers, used by Alembic.
revision = '1<PASSWORD>'
down_revision = '6<PASSWORD>'
branch_labels = None
depends_on = None
def upgrade():
# ### commands auto generated by Alembic - please adjust! ###
op.add_column('categorys', sa.Column('aid', sa.Integer(), nullable=True))
op.create_foreign_key(None, 'categorys', 'posts', ['aid'], ['id'])
op.drop_constraint('posts_ibfk_1', 'posts', type_='foreignkey')
op.drop_column('posts', 'cid')
# ### end Alembic commands ###
def downgrade():
# ### commands auto generated by Alembic - please adjust! ###
op.add_column('posts', sa.Column('cid', mysql.INTEGER(display_width=11), autoincrement=False, nullable=True))
op.create_foreign_key('posts_ibfk_1', 'posts', 'categorys', ['cid'], ['id'])
op.drop_constraint(None, 'categorys', type_='foreignkey')
op.drop_column('categorys', 'aid')
# ### end Alembic commands ###
| f5ca5de05ccaf7483a199e6ac2dc1e636b07e038 | [
"Markdown",
"Python",
"Text",
"INI"
] | 24 | Markdown | alone-zd/flask-blog | 2a173b3383286aa44aff2dce614ad291d4f80c7c | b739ea6afa108686a37c47dce04beb21089a930f | |
refs/heads/main | <repo_name>heydrigh/TGL-react<file_sep>/src/store/modules/lotterys/actions/actionTypes.js
export const FETCH_LOTTERY = 'FETCH_LOTTERY';
export const FETCH_LOTTERY_START = 'FETCH_LOTTERY_START';
export const FETCH_LOTTERY_SUCCESS = 'FETCH_LOTTERY_SUCCESS';
export const FETCH_LOTTERY_FAIL = 'FETCH_LOTTERY_FAIL';
<file_sep>/src/pages/Account/index.jsx
import React, { useEffect } from 'react';
import * as S from './styles';
import { AiOutlineArrowRight, AiOutlineArrowLeft } from 'react-icons/ai';
import { useSelector } from 'react-redux';
import { useHistory, Link } from 'react-router-dom';
import { useForm } from 'react-hook-form';
import { toast } from 'react-toastify';
import api from '../../services/api';
const Account = () => {
const { register, handleSubmit } = useForm();
const history = useHistory();
const auth = useSelector((state) => {
return {
isAuth: state.auth.isAuth,
user: state.auth.user
};
});
useEffect(() => {
if (!auth.isAuth) {
history.push('/');
}
}, [auth.isAuth, history]);
const onSubmit = async (data) => {
try {
const response = await api.put('/users', data);
console.log(response);
toast.success('Suas alterações foram salvas');
history.push('/dashboard');
} catch (err) {
console.log(err);
toast.error('Falha em modificar seus dados, tente novamente mais tarde');
}
};
return (
<S.Wrapper>
<S.Header>Account</S.Header>
<S.Card onSubmit={handleSubmit(onSubmit)}>
<S.InputsWrapper>
<label>Nome</label>
<input
name="username"
ref={register({ required: true })}
type="text"
placeholder={auth.user.username}
/>
<label>Email</label>
<input
name="email"
type="email"
placeholder={auth.user.email}
disabled
/>
<label>Senha</label>
<input
name="password"
ref={register({ required: true })}
type="password"
/>
<label>Confirmação de senha</label>
<input
name="password_confirmation"
ref={register({ required: true })}
type="password"
/>
</S.InputsWrapper>
<S.SubmitButton type="submit">
Modify <AiOutlineArrowRight size={30} />
</S.SubmitButton>
</S.Card>
<S.LastButton>
<Link to="/dashboard">
<AiOutlineArrowLeft size={30} />
Back
</Link>
</S.LastButton>
</S.Wrapper>
);
};
export default Account;
<file_sep>/src/store/modules/lotterys/actions/index.js
export {
fecthLottery,
fetchLotterySuccess,
fetchLotteryFail,
fetchLotteryStart
} from './actions';
<file_sep>/src/pages/NewGame/index.jsx
import React, { useEffect, useState } from 'react';
import { connect } from 'react-redux';
import * as S from './styles';
import Menu from '../../components/Menu';
import LotteryButton from '../../components/LotteryButton';
import Ball from '../../components/Ball';
import Cart from '../../components/Cart';
import * as lotteryActions from '../../store/modules/lotterys/actions/actions';
import * as betsActions from '../../store/modules/bets/actions/actions';
import Spinner from '../../components/Spinner';
import uuid from 'react-uuid';
import { toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
import { FiShoppingCart } from 'react-icons/fi';
import { useHistory } from 'react-router-dom';
const NewGame = (props) => {
const [selectableNumbers, setSelectableNumbers] = useState([]);
const [isActive, setIsActive] = useState([]);
const [chosenGame, setChosenGame] = useState('');
const [rules, setRules] = useState({});
const [cartsGames, setCartGames] = useState([]);
const [selectedNumbers, setSelectedNumbers] = useState([]);
const [instruction, setInstruction] = useState(
'Escolha um jogo para começar!'
);
const history = useHistory();
useEffect(() => {
props.onFecthLottery();
if (!props.auth.isAuth) {
history.push('/');
}
}, []);
const handleSelectedGame = (gameRange, gameInstruction, gameName) => {
let numbers = [];
let gameInfos = [];
for (let i = 1; i <= gameRange; i++) {
numbers.push(i);
}
setSelectableNumbers(numbers);
handleInstructions(gameInstruction);
Object.values(props.types).map((name) => {
gameInfos.push(name.type);
});
setChosenGame(gameName);
let filteredGame = Object.values(props.types).filter((game) => {
return game.type === gameName;
});
setRules(filteredGame[0]);
console.log(props.types);
};
const handleInstructions = (gameInstructions) => {
setInstruction(gameInstructions);
};
const handleBallClick = (number) => {
const numbers = [...selectedNumbers];
const actives = isActive;
numbers.push(number);
setSelectedNumbers(numbers);
actives[number - 1] = !actives[number - 1];
setIsActive(actives);
};
const completeGameHandler = () => {
let numbers = [];
let selected = selectedNumbers;
const actives = isActive;
for (let i = 1; i <= rules.range; i++) {
numbers.push(i);
}
if (!chosenGame) {
toast.error('Escolha um jogo');
return;
}
if (selectedNumbers.length === rules.max_number) {
toast.error('Número maximo de números ja escolhido');
} else {
while (selected.length < rules.max_number) {
const filteredNumbers = numbers.filter((item) => {
return !selected.includes(item);
});
let randomNumber =
filteredNumbers[Math.floor(Math.random() * filteredNumbers.length)];
selected.push(randomNumber);
actives[randomNumber - 1] = !actives[randomNumber - 1];
setIsActive(actives);
}
setSelectedNumbers([...selected]);
}
};
const handleRemoveBet = (betId) => {
setCartGames(cartsGames.filter((bet) => bet.id !== betId));
};
const addToCartHandler = () => {
if (selectedNumbers.length === rules.max_number) {
const gameDone = {
...rules,
selectedNumbers,
game_id: uuid()
};
gameDone.selectedNumbers.sort();
setCartGames([...cartsGames, gameDone]);
clearGameHandler();
toastSuccess('Jogo adicionado ao carrinho com sucesso!');
console.log(gameDone);
} else {
toastWarn(rules.max_number);
}
};
const clearGameHandler = () => {
const numbers = [];
setSelectedNumbers([]);
for (let i = 0; i < 80; i++) {
numbers.push(false);
}
setIsActive(numbers);
};
const toastWarn = (maxNumber) => {
toast.warn(`Por favor, escolha ${maxNumber} números para seu jogo`, {
position: 'top-right',
autoClose: 5000,
hideProgressBar: false,
closeOnClick: true,
pauseOnHover: true,
draggable: true,
progress: undefined
});
};
const toastSuccess = (message) => {
toast.info(message, {
position: 'top-right',
autoClose: 5000,
hideProgressBar: false,
closeOnClick: true,
pauseOnHover: true,
draggable: true,
progress: undefined
});
};
return (
<>
<Menu
homeText="Home"
homeLink="/dashboard"
accountText="Account"
accountLink="/account"
logOutText="Logout"
/>
<S.Wrapper>
<S.GamesWrapper>
<S.GameHeader>
<S.NewBet>New bet</S.NewBet>
{chosenGame.length < 1 ? (
<S.GameName>Click a game to begin</S.GameName>
) : (
<S.GameName>For {chosenGame}</S.GameName>
)}
</S.GameHeader>
<S.ChoiceWrapper>
<S.ChoiceHeader>Choose a game</S.ChoiceHeader>
{props.loading ? (
<Spinner />
) : (
<S.ChoicesButtons>
{props.types &&
Object.values(props.types).map((lotteryButton) => (
<LotteryButton
clicked={() =>
handleSelectedGame(
lotteryButton.range,
lotteryButton.description,
lotteryButton.type
)
}
key={lotteryButton.type}
lotteryName={lotteryButton.type}
color={lotteryButton.color}
active={lotteryButton.type === chosenGame}
/>
))}
</S.ChoicesButtons>
)}
</S.ChoiceWrapper>
<S.InstructionsWrapper>
<S.InstructionsHeader>Fill your bet</S.InstructionsHeader>
<S.Instructions>{instruction}</S.Instructions>
</S.InstructionsWrapper>
<S.NumbersWrapper>
{selectableNumbers &&
selectableNumbers.map((number) => (
<Ball
isActive={isActive[number - 1]}
disabled={isActive[number - 1]}
clicked={() => handleBallClick(number)}
number={number}
key={number}
color={rules.color}
/>
))}
</S.NumbersWrapper>
<S.ControlsWrapper>
<S.CompleteAndClear>
<button onClick={completeGameHandler}>Complete Game</button>
<button onClick={clearGameHandler}>Clear Game</button>
</S.CompleteAndClear>
<S.CartControl>
<button onClick={addToCartHandler}>
<FiShoppingCart size={24} />
Add to cart
</button>
</S.CartControl>
</S.ControlsWrapper>
</S.GamesWrapper>
<Cart bets={cartsGames} deleted={handleRemoveBet} />
</S.Wrapper>
</>
);
};
const mapStateToProps = (state) => {
return {
types: state.types.lottery[0],
loading: state.types.loading,
auth: state.auth
};
};
const mapDispatchToProps = (dispatch) => {
return {
onFecthLottery: () => dispatch(lotteryActions.fecthLottery()),
onSaveBet: (bets) => dispatch(betsActions.saveBetSuccess(bets))
};
};
export default connect(mapStateToProps, mapDispatchToProps)(NewGame);
<file_sep>/src/store/modules/bets/sagas/sagas.js
import api from '../../../../services/api';
import { put } from 'redux-saga/effects';
import * as actions from '../actions/actions';
export function* fetchBetsSaga(action) {
yield put(actions.fetchBetStart());
try {
const response = yield api.get('/games');
const fetchedGames = [];
fetchedGames.push({
...response.data
});
yield put(actions.fetchBetSuccess(fetchedGames));
} catch (error) {
yield put(actions.fetchBetFail(error));
}
}
<file_sep>/src/components/Cart/index.jsx
import React from 'react';
import * as S from './styles';
import { useHistory } from 'react-router-dom';
import { toast } from 'react-toastify';
import { BsTrash, BsArrowRight } from 'react-icons/bs';
import api from '../../services/api';
const Cart = ({ bets, deleted }) => {
const totalPrice = bets.reduce((acc, bets) => acc + bets.price, 0);
const history = useHistory();
const handleSavedButton = async () => {
let game_array = [];
if (bets.length > 0) {
bets.map((bet) => {
const game = {
type_id: bet.id,
numbers: bet.selectedNumbers.join(',')
};
game_array.push(game);
});
try {
const response = await api.post('/games', game_array);
toast.success('Seu jogos foras salvos com sucesso!');
console.log(response);
history.push('/dashboard');
} catch (err) {
console.log(err);
toast.error(
'Ocorreu um erro ao realizar esta ação, tente novamente mais tarde.'
);
}
} else {
toastWarn('Seu carrinho está vazio. Por favor faça ao menos uma aposta.');
}
};
const toastWarn = (message) => {
toast.warn(message, {
position: 'top-right',
autoClose: 5000,
hideProgressBar: false,
closeOnClick: true,
pauseOnHover: true,
draggable: true,
progress: undefined
});
};
return (
<>
<S.Wrapper>
<S.Cart>
<S.Header>CART</S.Header>
<S.BetsWrapper>
{bets.length > 0 ? (
bets.map((bet) => (
<S.Bet key={bet.id}>
<S.DeleteButton onClick={() => deleted(bet.id)}>
<BsTrash size={26} />
</S.DeleteButton>
<S.BetDetails color={bet.color}>
<S.BetNumbers>{bet.selectedNumbers.join()}</S.BetNumbers>
<S.NameAndPrice>
<S.LotteryName color={bet.color}>
{bet.type}
</S.LotteryName>
<S.LoteryPrice>
{' '}
{bet.price.toLocaleString('pt-br', {
style: 'currency',
currency: 'BRL'
})}
</S.LoteryPrice>
</S.NameAndPrice>
</S.BetDetails>
</S.Bet>
))
) : (
<span>Carrinho vazio. Faça uma aposta!</span>
)}
</S.BetsWrapper>
<S.CartTotal>
<strong>CART</strong> TOTAL:{' '}
{totalPrice.toLocaleString('pt-br', {
style: 'currency',
currency: 'BRL'
})}
</S.CartTotal>
</S.Cart>
<S.SaveButton onClick={handleSavedButton}>
Save <BsArrowRight size={38} />
</S.SaveButton>
</S.Wrapper>
</>
);
};
export default Cart;
<file_sep>/src/store/modules/bets/actions/actionTypes.js
export const FETCH_BET_START = 'FETCH_BET_START';
export const FETCH_BET_SUCCESS = 'FETCH_BET_SUCCESS';
export const FETCH_BET_FAIL = 'FETCH_BET_FAIL';
export const FETCH_BET = 'FETCH_BET';
export const SAVE_BET_START = 'SAVE_BET_START';
export const SAVE_BET_SUCCESS = 'SAVE_BET_SUCCESS';
export const SAVE_BET_FAIL = 'SAVE_BET_FAIL';
<file_sep>/src/components/CardForm/styles.js
import styled from 'styled-components';
export const Wrapper = styled.div`
display: flex;
flex-direction: column;
`;
export const Header = styled.h1`
text-align: center;
font: italic normal bold 35px Arial;
letter-spacing: 0px;
color: #707070;
margin-bottom: 25px;
`;
export const Card = styled.form`
display: flex;
flex-direction: column;
width: 352px;
background: #ffffff 0% 0% no-repeat padding-box;
box-shadow: 0px 3px 25px #00000014;
border: 1px solid #dddddd;
border-radius: 14px;
`;
export const InputsWrapper = styled.form`
display: flex;
flex: 1;
flex-direction: column;
div {
display: flex;
flex-direction: column;
label {
text-align: left;
font: italic normal bold 17px Arial;
margin-top: 34px;
margin-left: 30px;
letter-spacing: 0px;
color: #9d9d9d;
}
input {
flex: 1;
border-bottom: 2px solid #ebebeb;
color: #9d9d9d;
padding-left: 20px;
}
}
`;
export const OptionalLink = styled.span`
flex: 1;
text-align: right;
font: italic normal normal 17px Arial;
letter-spacing: 0px;
color: #c1c1c1;
margin-top: 27px;
margin-right: 27px;
a {
text-decoration: none;
color: #c1c1c1;
}
`;
export const SubmitButton = styled.button`
flex: 1;
text-align: center;
font: italic normal bold 35px Arial;
letter-spacing: 0px;
color: #b5c401;
background: none;
margin-top: 44px;
margin-bottom: 43px;
svg {
vertical-align: middle;
}
a {
text-decoration: none;
color: #b5c401;
}
`;
export const LastButton = styled.span`
flex: 1;
text-align: center;
font: italic normal bold 35px Arial;
letter-spacing: 0px;
color: #707070;
margin-top: 30px;
a {
text-decoration: none;
color: #707070;
}
`;
<file_sep>/src/pages/NewGame/styles.js
import styled, { keyframes } from 'styled-components';
import media from 'styled-media-query';
import { shade, lighten } from 'polished';
const appearFromLeft = keyframes`
from {
opacity: 0;
transform: translateX(-50px);
}
to {
opacity: 1;
transform: translateX(0);
}
`;
export const Wrapper = styled.div`
display: flex;
align-self: center;
justify-content: center;
flex-direction: row;
margin-top: 72px;
align-items: center;
overflow-x: hidden;
animation: ${appearFromLeft} 1s;
${media.lessThan('medium')`
margin-top: 70px;
flex-direction: column;
`}
`;
export const GamesWrapper = styled.div`
width: 1080px;
display: flex;
align-self: center;
flex-direction: column;
${media.lessThan('medium')`
margin-bottom: 20px;
margin-left: 400px;
margin: auto;
padding: 20px;
`}
`;
export const GameHeader = styled.div`
display: flex;
text-align: left;
text-transform: uppercase;
${media.lessThan('medium')`
flex-direction: row;
margin-bottom: 20px;
`}
`;
export const NewBet = styled.h1`
text-align: center;
font: italic normal bold 24px Arial;
letter-spacing: 0px;
color: #707070;
margin-right: 10px;
${media.lessThan('medium')`
flex-direction: row;
`}
`;
export const GameName = styled.h1`
text-align: center;
font: italic normal 300 24px Arial;
letter-spacing: 0px;
color: #707070;
${media.lessThan('medium')`
flex-direction: row;
margin-bottom: 20px;
`}
`;
export const ChoiceWrapper = styled.div`
display: flex;
flex-direction: column;
margin-top: 33px;
${media.lessThan('medium')`
flex-direction: row;
margin-bottom: 20px;
`}
`;
export const ChoiceHeader = styled.div`
text-align: left;
font: italic normal bold 17px Arial;
letter-spacing: 0px;
color: #868686;
${media.lessThan('medium')`
text-align: center;
`}
`;
export const ChoicesButtons = styled.div`
display: flex;
margin-top: 20px;
${media.lessThan('medium')`
flex-direction: column;
margin-bottom: 20px;
margin-top: 20px;
`}
`;
export const InstructionsWrapper = styled.div`
margin-top: 37px;
`;
export const InstructionsHeader = styled.p`
font: italic normal bold 17px Arial;
letter-spacing: 0px;
`;
export const Instructions = styled.p`
width: 65%;
font: italic normal normal 17px Arial;
letter-spacing: 0px;
`;
export const NumbersWrapper = styled.div`
display: grid;
grid-template-columns: repeat(10, 1fr);
gap: 20px;
margin-top: 25px;
width: 60%;
margin-bottom: 44px;
${media.lessThan('medium')`
grid-template-columns: repeat(6, 1fr);
gap: 5px;
width: 200px;
`}
`;
export const ControlsWrapper = styled.div`
width: 60%;
display: flex;
justify-content: space-around;
${media.lessThan('medium')`
width: 400px;
justify-content: center;
align-items: center;
flex-direction: column;
`}
`;
export const CompleteAndClear = styled.div`
button {
height: 52px;
border: 1px solid #27c383;
border-radius: 10px;
font: normal normal medium 16px Arial;
letter-spacing: 0px;
color: #27c383;
background: none;
transition: background-color 0.4s;
padding: 8px;
&:hover {
background-color: ${shade(0.2, '#27c383')};
color: #fff;
}
& + button {
margin-left: 25px;
}
}
${media.lessThan('medium')`
margin-bottom: 10px;
`}
`;
export const CartControl = styled.div`
button {
width: 209px;
height: 52px;
background: #27c383 0% 0% no-repeat padding-box;
border: 1px solid #27c383;
border-radius: 10px;
font: normal normal bold 16px Arial;
letter-spacing: 0px;
color: #ffffff;
transition: background-color 0.4s;
&:hover {
background-color: ${lighten(0.05, '#27c383')};
color: #fff;
}
}
svg {
vertical-align: middle;
margin-right: 28px;
color: #fff;
}
`;
<file_sep>/src/store/modules/lotterys/sagas/sagas.js
import api from '../../../../services/api';
import { put } from 'redux-saga/effects';
import * as actions from '../actions/actions';
export function* fetchedLotterySaga(action) {
yield put(actions.fetchLotteryStart());
try {
const response = yield api.get('/types');
const fetchedLottery = [];
fetchedLottery.push({
...response.data
});
yield put(actions.fetchLotterySuccess(fetchedLottery));
} catch (error) {
yield put(actions.fetchLotteryFail(error));
}
}
<file_sep>/src/store/modules/bets/reducers/reducer.js
import * as actionTypes from '../actions/actionTypes';
import { updateObject } from '../../../utility';
const initialState = {
bets: [],
loading: true,
error: false
};
const saveBet = (state, action) => {
const updatedState = {
bets: action.bets.concat(state.bets)
};
return updateObject(state, updatedState);
};
const fetchBetStart = (state, action) => {
return updateObject(state, { loading: true });
};
const fetchBetFail = (state, action) => {
return updateObject(state, { loading: false });
};
const fetchBetFinished = (state, action) => {
return updateObject(state, { loading: false });
};
const fetchBets = (state, action) => {
return updateObject(state, {
bets: action.bets
});
};
const reducer = (state = initialState, action) => {
switch (action.type) {
case actionTypes.SAVE_BET_SUCCESS:
return saveBet(state, action);
case actionTypes.FETCH_BET_START:
return fetchBetStart(state, action);
case actionTypes.FETCH_BET_FAIL:
return fetchBetFail(state, action);
case actionTypes.FETCH_BET_SUCCESS:
return fetchBets(state, action);
default:
return state;
}
};
export default reducer;
<file_sep>/src/pages/ResetPassword/index.jsx
import React from 'react';
import * as S from './styles';
import Header from '../../components/Header';
import CardForm from '../../components/CardForm';
import Footer from '../../components/Footer';
const ResetPassword = () => {
const inputFields = [
{
label: 'Email',
type: 'text',
name: 'email'
}
];
return (
<>
<S.Wrapper>
<Header />
<CardForm
header="Reset password"
inputFields={inputFields}
submitButtonText="Send link"
lastButtonLink="/"
lastButtonText="Back"
methor="forgot"
/>
</S.Wrapper>
<Footer />
</>
);
};
export default ResetPassword;
<file_sep>/src/store/modules/lotterys/actions/actions.js
import * as actionTypes from './actionTypes';
export const fetchLotteryStart = () => {
return {
type: actionTypes.FETCH_LOTTERY_START
};
};
export const fetchLotterySuccess = (lottery) => {
return {
type: actionTypes.FETCH_LOTTERY_SUCCESS,
lottery: lottery
};
};
export const fetchLotteryFail = (error) => {
return {
type: actionTypes.FETCH_LOTTERY_FAIL,
error: error
};
};
export const fecthLottery = () => {
return {
type: actionTypes.FETCH_LOTTERY
};
};
<file_sep>/src/components/LotteryButton/styles.js
import styled, { css } from 'styled-components';
import { shade } from 'polished';
import media from 'styled-media-query';
export const Wrapper = styled.button`
width: 113px;
height: 34px;
background: #ffffff 0% 0% no-repeat padding-box;
margin-right: 25px;
border-radius: 100px;
text-align: center;
font: italic normal bold 14px Arial;
letter-spacing: 0px;
transition: background-color 0.2s;
${media.lessThan('medium')`
margin-bottom: 10px;
;
`}
${(props) =>
props.color &&
css`
color: ${props.color};
border: 2px solid ${props.color};
&:hover {
background: ${shade(0.1, `${props.color}`)};
color: #ffffff;
}
`}
${(props) =>
props.active &&
css`
color: ${props.color};
background: ${shade(0.1, `${props.color}`)};
color: #ffffff;
`}
`;
<file_sep>/src/styles/themes.js
export default {
font: {
family:
"Arial ,Roboto, Ubuntu, 'Open Sans', sans-serif",
bold: 600,
},
colors: {
primary: '##F7F7F7',
primaryHover: '#ADC0C4',
lotofacil: '#7F3992',
megasena: '#10AC66',
lotomania: '#FAAE31',
cartbg: '#FFFFFF',
boxBg: '#FAFAFA',
texts: '#707070',
white: '#FFFFFF',
black: '#000000',
gray: '#7B7373'
},
}
<file_sep>/src/pages/Dashboard/styles.js
import styled, { keyframes } from 'styled-components';
import media from 'styled-media-query';
const appearFromLeft = keyframes`
from {
opacity: 0;
transform: translateX(-50px);
}
to {
opacity: 1;
transform: translateX(0);
}
`;
export const Wrapper = styled.div`
display: flex;
width: 70%;
flex-direction: column;
align-items: flex-start;
margin-left: 390px;
margin-top: 75px;
animation: ${appearFromLeft} 1s;
${media.lessThan('medium')`
margin-bottom: 20px;
margin: auto;
`}
`;
export const GamesHeader = styled.div`
display: flex;
${media.lessThan('medium')`
justify-content: center;
align-items: center;
`}
`;
export const Recent = styled.h4`
font: italic normal bold 24px Arial;
letter-spacing: 0px;
color: #707070;
text-transform: uppercase;
`;
export const FilterWrapper = styled.div`
display: flex;
margin-left: 46px;
${media.lessThan('medium')`
flex-direction: column;
margin-left: 0;
`}
`;
export const FilterSpan = styled.span`
font: italic normal normal 17px Arial;
letter-spacing: 0px;
padding-top: 4px;
color: #868686;
${media.lessThan('medium')`
text-align: center;
margin-left: -6px;
margin-bottom: 4px;
`}
`;
export const FiltersContainer = styled.div`
margin-left: 16px;
`;
export const NewBet = styled.div`
font: italic normal bold 24px Arial;
letter-spacing: 0px;
margin-left: 200px;
a {
color: #b5c401;
text-decoration: none;
}
svg {
vertical-align: middle;
}
${media.lessThan('medium')`
margin-left: 0;
`}
`;
export const GamesWrapper = styled.div`
margin-top: 38px;
span {
font: normal normal normal 20px Arial;
letter-spacing: 0px;
color: #868686;
}
${media.lessThan('medium')`
left: 0;
`}
`;
export const Game = styled.div`
display: flex;
margin-bottom: 30px;
hr {
border: 3px solid ${(props) => props.color && props.color};
border-radius: 10px;
margin-right: 15px;
}
`;
export const GameDetails = styled.div``;
export const GameNumbers = styled.div`
font: italic normal bold 20px Arial;
letter-spacing: 0px;
color: #868686;
margin-bottom: 15px;
`;
export const DateAndCost = styled.div`
font: normal normal normal 17px Arial;
letter-spacing: 0px;
color: #868686;
margin-bottom: 11px;
`;
export const GameName = styled.div`
font: italic normal bold 20px Arial;
letter-spacing: 0px;
color: ${(props) => props.color && props.color};
`;
<file_sep>/src/routes/index.jsx
import React from 'react';
import { Switch, Route } from 'react-router-dom';
import Home from '../pages/Home';
import ResetPassword from '../pages/ResetPassword';
import Register from '../pages/Register';
import NewGame from '../pages/NewGame';
import Dashboard from '../pages/Dashboard';
import Account from '../pages/Account';
const Routes = () => (
<Switch>
<Route path="/" exact component={Home} />
<Route path="/dashboard" component={Dashboard} />
<Route path="/account" component={Account} />
<Route path="/resetpassword" component={ResetPassword} />
<Route path="/register" component={Register} />
<Route path="/newgame" component={NewGame} />
</Switch>
);
export default Routes;
<file_sep>/src/components/Footer/styles.js
import styled from 'styled-components';
import media from 'styled-media-query';
export const Wrapper = styled.footer`
position: absolute;
bottom: 0;
width: 100%;
height: 50px;
display: flex;
justify-content: center;
align-items: center;
color: #707070;
border-top: 2px solid #ebebeb;
border-bottom: 2px solid #ebebeb;
font-size: 15px;
${media.lessThan('medium')`
display: none;
`}
`;
<file_sep>/src/pages/Register/index.jsx
import React from 'react';
import * as S from './styles';
import CardForm from '../../components/CardForm';
import Header from '../../components/Header';
import Footer from '../../components/Footer';
const Register = () => {
const inputFields = [
{
label: 'Name',
type: 'text',
name: 'username'
},
{
label: 'Email',
type: 'email',
name: 'email'
},
{
label: 'Password',
type: '<PASSWORD>',
name: '<PASSWORD>'
},
{
label: 'Password Confirmation',
type: 'password',
name: 'password_confirmation'
}
];
return (
<>
<S.Wrapper>
<Header />
<CardForm
header="Registration"
inputFields={inputFields}
submitButtonText="Register"
lastButtonLink="/"
lastButtonText="Back"
method="signUp"
/>
</S.Wrapper>
<Footer />
</>
);
};
export default Register;
<file_sep>/src/store/modules/bets/actions/actions.js
import * as actionTypes from './actionTypes';
export const saveBetStart = () => {
return {
type: actionTypes.SAVE_BET_START
};
};
export const saveBetSuccess = (bets) => {
return {
type: actionTypes.SAVE_BET_SUCCESS,
bets: bets
};
};
export const saveBetFail = (error) => {
return {
type: actionTypes.SAVE_BET_FAIL,
error: error
};
};
export const fetchBetStart = (token) => {
return {
type: actionTypes.FETCH_BET_START,
token
};
};
export const fetchBetSuccess = (bets) => {
return {
type: actionTypes.FETCH_BET_SUCCESS,
bets: bets,
loading: false
};
};
export const fetchBetFail = (error) => {
return {
type: actionTypes.FETCH_BET_FAIL,
error: error
};
};
export const fecthBet = () => {
return {
type: actionTypes.FETCH_BET
};
};
<file_sep>/src/components/Ball/index.jsx
import React from 'react';
import * as S from './styles';
const Ball = ({ number, clicked, isActive, disabled, color }) => {
return (
<S.Wrapper
color={color}
disabled={disabled}
isActive={isActive}
onClick={clicked}
>
<span>{number}</span>
</S.Wrapper>
);
};
export default Ball;
<file_sep>/src/store/modules/bets/sagas/index.js
import { takeEvery } from 'redux-saga/effects';
import * as actionTypes from '../actions/actionTypes';
import { fetchBetsSaga } from './sagas';
export function* watchBets() {
yield takeEvery(actionTypes.FETCH_BET, fetchBetsSaga);
}
<file_sep>/src/components/Menu/styles.js
import styled from 'styled-components';
import media from 'styled-media-query';
export const Wrapper = styled.nav`
display: flex;
top: 0;
padding-top: 15px;
padding-left: 141px;
padding-right: 200px;
height: 76px;
width: 100vw;
border-bottom: 2px solid #ebebeb;
justify-content: space-around;
${media.lessThan('medium')`
margin-left: 12px;
`}
`;
export const LogoAndHome = styled.div`
display: flex;
`;
export const Logo = styled.div`
text-align: center;
font: italic normal bold 44px Arial;
letter-spacing: 0px;
color: #707070;
hr {
border: 4px solid #b5c401;
border-radius: 6px;
width: 106px;
margin-top: 6px;
}
`;
export const Home = styled.div`
justify-content: center;
align-items: center;
text-align: center;
font: italic normal bold 20px Arial;
letter-spacing: 0px;
margin-left: 74px;
margin-top: 16px;
${media.lessThan('medium')`
margin-left: 10px;
margin-top: 10px;
`}
a {
color: #707070;
text-decoration: none;
}
`;
export const AccountAndLogout = styled.div`
padding-top: 10px;
span {
text-align: center;
font: italic normal bold 20px Arial;
letter-spacing: 0px;
color: #707070;
}
a {
text-align: center;
font: italic normal bold 20px Arial;
letter-spacing: 0px;
color: #707070;
text-decoration: none;
margin-left: 40px;
svg {
vertical-align: middle;
${media.lessThan('medium')`
display: none;
`}
}
}
${media.lessThan('medium')`
margin-left: 10px;
`}
`;
export const LogoutButton = styled.button`
text-align: center;
font: italic normal bold 20px Arial;
letter-spacing: 0px;
color: #707070;
text-decoration: none;
margin-left: 40px;
background: none;
svg {
vertical-align: middle;
${media.lessThan('medium')`
display: none;
`}
}
`;
<file_sep>/src/pages/Home/index.jsx
import React, { useEffect } from 'react';
import * as S from './styles';
import CardForm from '../../components/CardForm';
import Header from '../../components/Header';
import Footer from '../../components/Footer';
import { useHistory } from 'react-router-dom';
import { useSelector } from 'react-redux';
import { toast } from 'react-toastify';
const Home = () => {
const history = useHistory();
const auth = useSelector((state) => {
return {
isAuth: state.auth.isAuth,
error: state.auth.error
};
});
useEffect(() => {
if (auth.isAuth) {
history.push('/dashboard');
}
if (auth.error) {
toast.error(
'Algo deu errado ao fazer login, verifique seu email e senha.'
);
}
}, [auth.isAuth, history, auth]);
const inputFields = [
{
label: 'Email',
type: 'text',
name: 'email'
},
{
label: 'Password',
type: 'password',
name: 'password'
}
];
return (
<>
<S.Wrapper>
<Header />
<CardForm
header="Authentication"
optionalLink="/resetpassword"
optionalText="I forgot my password"
inputFields={inputFields}
submitButtonText="Log In"
submitButtonLink="/dashboard"
lastButtonLink="/register"
lastButtonText="Sign Up"
lastIconRight={true}
method="login"
/>
</S.Wrapper>
<Footer />
</>
);
};
export default Home;
<file_sep>/src/store/modules/auth/sagas/index.js
import { takeEvery } from 'redux-saga/effects';
import * as actionTypes from '../actions/actionTypes';
import { authSagas } from './sagas';
export function* watchAuth() {
yield takeEvery(actionTypes.AUTH_REQUEST, authSagas);
}
<file_sep>/src/pages/Register/styles.js
import styled, { keyframes } from 'styled-components';
import media from 'styled-media-query';
const appearFromRight = keyframes`
from {
opacity: 0;
transform: translateX(50px);
}
to {
opacity: 1;
transform: translateX(0);
}
`;
export const Wrapper = styled.div`
display: flex;
flex-direction: row;
margin-top: 154px;
align-items: center;
justify-content: space-evenly;
overflow-x: hidden;
animation: ${appearFromRight} 1s;
${media.lessThan('medium')`
flex-direction: column;
margin-top: 70px;
`}
`;
<file_sep>/src/components/Ball/styles.js
import styled from 'styled-components';
import { shade } from 'polished';
export const Wrapper = styled.button`
display: flex;
justify-content: center;
align-items: center;
border-radius: 50%;
width: 65px;
height: 65px;
font: normal normal bold 20px Arial;
letter-spacing: 0px;
color: #ffffff;
transition: cursor 0.2s;
background: ${(props) => (props.isActive ? props.color : '#adc0c4;')};
/* &:hover {
background: ${shade(0.4, '#adc0c4')};
cursor: pointer;
} */
&:disabled {
cursor: not-allowed;
:hover {
background-color: ${(props) => props.color && props.color};
}
}
`;
| 7c384f0181497ab1e16ddc96bd1f6a48eeab351b | [
"JavaScript"
] | 27 | JavaScript | heydrigh/TGL-react | 27cf6d74e0253a2b2c2df1cc7bfcf3e63655f2e4 | 69e30eed8b5dd17ae05dc8c7766ce5d8f9af73da | |
refs/heads/master | <repo_name>bianchengxiaosheng/Unreal4-Dynami-Umg-Test<file_sep>/AutoUI/Plugins/AutoGenerateUMGByXML/Source/AutoGenerateUMGByXML/Private/GenerateUMGFactory.cpp
// Fill out your copyright notice in the Description page of Project Settings.
#include "GenerateUMGFactory.h"
#include "Classes/WidgetBlueprintFactory.h"
#include "Blueprint/UserWidget.h"
#include "Runtime/CoreUObject//Public/UObject/Package.h"
#include "Runtime/AssetRegistry/Public/AssetRegistryModule.h"
#include "Editor/UMGEditor/Public/WidgetBlueprint.h"
#include "Runtime/UMG/Public/UMG.h"
#include "Public/CoreUObject.h"
//class UMG;
//class Factory;
//class UCanvasPanel;
//class UImage;
//class UTextBlock;
//class UWidgetTree;
DEFINE_LOG_CATEGORY_STATIC(LogGenerateUMGFactory, Log, All);
GenerateUMGFactory::GenerateUMGFactory()
{
}
GenerateUMGFactory::~GenerateUMGFactory()
{
}
void GenerateUMGFactory::GenerateUMGByXML()
{
UE_LOG(LogGenerateUMGFactory, Warning, TEXT("GenerateUMGByXML"));
//NewWisget
UFactory *factory = NewObject<UWidgetBlueprintFactory>();
char * name = "/Game/W_MyWidgetBlueprint";
UPackage *outer = CreatePackage(nullptr, UTF8_TO_TCHAR(name));
UClass *u_class = factory->GetSupportedClass();
char *obj_name = strrchr(name, '/') + 1;
if (u_class->IsChildOf<UBlueprint>())
{
if (FindObject<UBlueprint>(outer, UTF8_TO_TCHAR(obj_name)))
{
}
}
UObject *u_object = factory->FactoryCreateNew(u_class, outer, FName(UTF8_TO_TCHAR(obj_name)), RF_Public | RF_Standalone, nullptr, GWarn);
FAssetRegistryModule::AssetCreated(u_object);
outer->MarkPackageDirty();
UWidgetBlueprint* widget = Cast<UWidgetBlueprint>(u_object);
widget->Modify();
UCanvasPanel* slot1 = widget->WidgetTree->ConstructWidget<UCanvasPanel>(UCanvasPanel::StaticClass(), FName(TEXT("Slot1")));
UImage* image1 = widget->WidgetTree->ConstructWidget<UImage>(UImage::StaticClass(),
FName(TEXT("image1")));
slot1->AddChild(image1);
Cast<UPanelWidget>(slot1)->AddChild(image1);
//UCanvasPanelSlot *slottemp = new UCanvasPanelSlot>("",widget->WidgetTree);
//slottemp->Content = image1;
//UCanvasPanelSlot* slot2 = widget->WidgetTree->ConstructWidget<UCanvasPanelSlot>(UCanvasPanelSlot::StaticClass(),
// FName(TEXT("Slot1")));
UTextBlock* text_block = widget->WidgetTree->ConstructWidget<UTextBlock>(UTextBlock::StaticClass(),
FName(TEXT("text_block")));
text_block->Text = FText::FromString(FString("Hellow"));
//slot2->Content = text_block;
UPanelWidget* rootWidgetTemp = Cast<UPanelWidget>(widget->WidgetTree->RootWidget);
rootWidgetTemp->ClearChildren();
rootWidgetTemp->AddChild(slot1);
rootWidgetTemp->AddChild(image1);
rootWidgetTemp->AddChild(text_block);
widget->PostEditChange();
}
<file_sep>/README.md
# Unreal4-Dynami-Umg-Test
Unreal4 Dynami Umg Test
<file_sep>/AutoUI/Plugins/AutoGenerateUMGByXML/Source/AutoGenerateUMGByXML/Public/GenerateUMGFactory.h
// Fill out your copyright notice in the Description page of Project Settings.
#pragma once
#include "CoreMinimal.h"
/**
*
*/
class AUTOGENERATEUMGBYXML_API GenerateUMGFactory
{
public:
GenerateUMGFactory();
~GenerateUMGFactory();
void GenerateUMGByXML();
};
<file_sep>/AutoUI/Source/AutoUI/MyUserWidget.cpp
// Fill out your copyright notice in the Description page of Project Settings.
#include "MyUserWidget.h"
//void UMyUserWidget::NativeConstruct()
//{
// Super::NativeConstruct();
//
//}
void UMyUserWidget::OnCreationFromPalette()
{
}
<file_sep>/AutoUI/Plugins/AutoGenerateUMGByXML/Source/AutoGenerateUMGByXML/Private/AutoGenerateUMGByXMLCommands.cpp
// Copyright 1998-2018 Epic Games, Inc. All Rights Reserved.
#include "AutoGenerateUMGByXMLCommands.h"
#define LOCTEXT_NAMESPACE "FAutoGenerateUMGByXMLModule"
void FAutoGenerateUMGByXMLCommands::RegisterCommands()
{
UI_COMMAND(PluginAction, "AutoGenerateUMGByXML", "Execute AutoGenerateUMGByXML action", EUserInterfaceActionType::Button, FInputGesture());
}
#undef LOCTEXT_NAMESPACE
<file_sep>/AutoUI/Plugins/AutoGenerateUMGByXML/Source/AutoGenerateUMGByXML/Private/AutoGenerateUMGByXML.cpp
// Copyright 1998-2018 Epic Games, Inc. All Rights Reserved.
#include "AutoGenerateUMGByXML.h"
#include "AutoGenerateUMGByXMLStyle.h"
#include "AutoGenerateUMGByXMLCommands.h"
#include "Misc/MessageDialog.h"
#include "Framework/MultiBox/MultiBoxBuilder.h"
#include "LevelEditor.h"
static const FName AutoGenerateUMGByXMLTabName("×Ô¶¯Éú³ÉUMG");
#define LOCTEXT_NAMESPACE "FAutoGenerateUMGByXMLModule"
DEFINE_LOG_CATEGORY_STATIC(LogAutoGenerateUMG, Log, All);
void FAutoGenerateUMGByXMLModule::StartupModule()
{
// This code will execute after your module is loaded into memory; the exact timing is specified in the .uplugin file per-module
umgFactory = new GenerateUMGFactory();
FAutoGenerateUMGByXMLStyle::Initialize();
FAutoGenerateUMGByXMLStyle::ReloadTextures();
FAutoGenerateUMGByXMLCommands::Register();
PluginCommands = MakeShareable(new FUICommandList);
PluginCommands->MapAction(
FAutoGenerateUMGByXMLCommands::Get().PluginAction,
FExecuteAction::CreateRaw(this, &FAutoGenerateUMGByXMLModule::PluginButtonClicked),
FCanExecuteAction());
FLevelEditorModule& LevelEditorModule = FModuleManager::LoadModuleChecked<FLevelEditorModule>("LevelEditor");
//
//{
// TSharedPtr<FExtender> MenuExtender = MakeShareable(new FExtender());
// MenuExtender->AddMenuExtension("WindowLayout", EExtensionHook::After, PluginCommands, FMenuExtensionDelegate::CreateRaw(this, &FAutoGenerateUMGByXMLModule::AddMenuExtension));
// LevelEditorModule.GetMenuExtensibilityManager()->AddExtender(MenuExtender);
//}
//
//{
// TSharedPtr<FExtender> ToolbarExtender = MakeShareable(new FExtender);
// ToolbarExtender->AddToolBarExtension("Settings", EExtensionHook::After, PluginCommands, FToolBarExtensionDelegate::CreateRaw(this, &FAutoGenerateUMGByXMLModule::AddToolbarExtension));
//
// LevelEditorModule.GetToolBarExtensibilityManager()->AddExtender(ToolbarExtender);
//}
{
TSharedPtr<FExtender> MenuBarExtender = MakeShareable(new FExtender);
MenuBarExtender->AddMenuBarExtension("Help", EExtensionHook::After, PluginCommands, FMenuBarExtensionDelegate::CreateRaw(this, &FAutoGenerateUMGByXMLModule::AddMenuBarExtension));
LevelEditorModule.GetMenuExtensibilityManager()->AddExtender(MenuBarExtender);
}
}
void FAutoGenerateUMGByXMLModule::ShutdownModule()
{
// This function may be called during shutdown to clean up your module. For modules that support dynamic reloading,
// we call this function before unloading the module.
FAutoGenerateUMGByXMLStyle::Shutdown();
FAutoGenerateUMGByXMLCommands::Unregister();
}
void FAutoGenerateUMGByXMLModule::PluginButtonClicked()
{
//UE_LOG(LogAutoGenerateUMG, Warning, TEXT("LogAutoGenerateUMG"));
umgFactory->GenerateUMGByXML();
// Put your "OnButtonClicked" stuff here
//FText DialogText = FText::Format(
// LOCTEXT("PluginButtonDialogText", "Add code to {0} in {1} to override this button's actions"),
// FText::FromString(TEXT("FAutoGenerateUMGByXMLModule::PluginButtonClicked()")),
// FText::FromString(TEXT("AutoGenerateUMGByXML.cpp"))
// );
//FMessageDialog::Open(EAppMsgType::Ok, DialogText);
}
void FAutoGenerateUMGByXMLModule::AddMenuExtension(FMenuBuilder& Builder)
{
Builder.AddMenuEntry(FAutoGenerateUMGByXMLCommands::Get().PluginAction);
}
void FAutoGenerateUMGByXMLModule::AddMenuBarExtension(FMenuBarBuilder &Builder)
{
Builder.AddPullDownMenu(
LOCTEXT("Custom_Menu_bar", "GenerateUMGByXML"),
LOCTEXT("Custom tip", "GenerateUMGByXML"),
FNewMenuDelegate::CreateRaw(this, &FAutoGenerateUMGByXMLModule::NewMenuBar),
"GenerateUMGByXML"
);
}
void FAutoGenerateUMGByXMLModule::NewMenuBar(FMenuBuilder &Builder) {
Builder.AddMenuEntry(FAutoGenerateUMGByXMLCommands::Get().PluginAction);
}
void FAutoGenerateUMGByXMLModule::AddToolbarExtension(FToolBarBuilder& Builder)
{
Builder.AddToolBarButton(FAutoGenerateUMGByXMLCommands::Get().PluginAction);
}
#undef LOCTEXT_NAMESPACE
IMPLEMENT_MODULE(FAutoGenerateUMGByXMLModule, AutoGenerateUMGByXML)<file_sep>/AutoUI/Source/AutoUI/AutoUIWidget.h
// Fill out your copyright notice in the Description page of Project Settings.
#pragma once
#include "CoreMinimal.h"
#include "Blueprint/UserWidget.h"
#include "Runtime/UMG/Public/UMG.h"
#include "Runtime/CoreUObject//Public/UObject/Package.h"
#include "Runtime/AssetRegistry/Public/AssetRegistryModule.h"
#include "AutoUIWidget.generated.h"
/**
*
*/
UCLASS()
class AUTOUI_API UAutoUIWidget : public UUserWidget
{
GENERATED_BODY()
public:
UPROPERTY(VisibleAnywhere, BlueprintReadWrite, Category = "UI")
UButton* StartButton;
UPROPERTY(VisibleAnywhere, BlueprintReadWrite, Category = "UI")
UButton* RegisterButton;
UPROPERTY(VisibleAnywhere, BlueprintReadWrite, Category = "UI")
UButton* ExitButton;
virtual void NativeConstruct() override;
//UPROPERTY(EditAnywhere, BlueprintReadWrite, meta = (BindWidgetOptional))
//UPROPERTY(VisibleAnywhere, BlueprintReadWrite, meta = (BindWidget))
UTextBlock * testTxtBlock;
////UButton* exampleButtton;
//
virtual TSharedRef<SWidget> RebuildWidget();
UComboBoxString* ComboServer;
UEditableTextBox* TxtBoxAccount;
UEditableTextBox* TxtBoxPwd;
bool Initialize() override;
UAutoUIWidget(const FObjectInitializer& ObjectInitializer);
//UPROPERTY(VisibleAnywhere, BlueprintReadWrite, Category = "UI")
UTextBlock* TxtDebugMsg;
//UFUNCTION()
// void ExitButClickEvent();
//UPROPERTY(EditAnywhere, BlueprintReadWrite, meta = (BindWidget))
UEditableText* editTxt;
//UPROPERTY(EditAnywhere, BlueprintReadWrite, meta = (BindWidget))
UCanvasPanel* RootPanel;
void InitTest();
#if WITH_EDITOR
virtual void OnCreationFromPalette() override;
#endif
private:
};
<file_sep>/AutoUI/Source/AutoUI/AutoUI.cpp
// Fill out your copyright notice in the Description page of Project Settings.
#include "AutoUI.h"
#include "Modules/ModuleManager.h"
IMPLEMENT_PRIMARY_GAME_MODULE( FDefaultGameModuleImpl, AutoUI, "AutoUI" );
<file_sep>/AutoUI/Source/AutoUI/AutoUIWidget.cpp
// Fill out your copyright notice in the Description page of Project Settings.
#include "AutoUIWidget.h"
#if WITH_EDITOR
#include "Editor/UMGEditor/Classes/WidgetBlueprintFactory.h"
#include "Editor/UnrealEd/Classes/Factories/Factory.h"
#include "Editor/UMGEditor/Public/WidgetBlueprint.h"
#endif
UAutoUIWidget::UAutoUIWidget(const FObjectInitializer& ObjectInitializer):Super(ObjectInitializer)
{
ComboServer = NULL;
TxtBoxAccount = nullptr;
TxtBoxPwd = nullptr;
}
bool UAutoUIWidget::Initialize()
{
if (!Super::Initialize())
{
return false;
}
if (UComboBoxString* combo = Cast<UComboBoxString>(GetWidgetFromName("ComboServer")))
{
ComboServer = combo;
}
if (UEditableTextBox* txtbox = Cast<UEditableTextBox>(GetWidgetFromName("TxtBoxAccount")))
{
TxtBoxAccount = txtbox;
TxtBoxAccount->SetKeyboardFocus();
}
if (UEditableTextBox* txtbox = Cast<UEditableTextBox>(GetWidgetFromName("TxtBoxPwd")))
{
TxtBoxPwd = txtbox;
}
/** 初始化游戏开始按钮 GetWidgetFromName 从实例化的蓝图中获取对应名字的控件*/
StartButton = Cast<UButton>(GetWidgetFromName(TEXT("Button_Start")));
/** 初始化游戏注册按钮 */
RegisterButton = Cast<UButton>(GetWidgetFromName(TEXT("Button_Register")));
/** 初始化游戏退出按钮 */
ExitButton = Cast<UButton>(GetWidgetFromName(TEXT("Button_Exit")));
/** 设置退出游戏按钮的点击事件 */
//if (ExitButton)
//{
// ExitButton->OnClicked.AddDynamic(this, &UAutoUIWidget::ExitButClickEvent);
//}
return true;
}
#if WITH_EDITOR
void UAutoUIWidget::OnCreationFromPalette()
{
}
#endif
void UAutoUIWidget::NativeConstruct()
{
Super::NativeConstruct();
//TxtDebugMsg = Cast<UTextBlock>(GetWidgetFromName(TEXT("TxtDebugMsg")));
}
void UAutoUIWidget::InitTest()
{
UPanelWidget* RootWidget = Cast<UPanelWidget>(GetRootWidget());
if (!RootWidget)
{
RootPanel = WidgetTree->ConstructWidget<UCanvasPanel>(UCanvasPanel::StaticClass(), FName(TEXT("RootPanel")));
RootWidget = RootPanel;
//UImage* image = WidgetTree->ConstructWidget<UImage>(UImage::StaticClass());
////image->SetBrushFromTexture(IndicatorTexture);
//image->SetColorAndOpacity(FLinearColor(0, 0, 0, 0));
//UPanelSlot* slot = RootWidget->AddChild(image);
//UCanvasPanelSlot* canvasSlot = Cast<UCanvasPanelSlot>(slot);
//canvasSlot->SetAnchors(FAnchors(0.5f, 0.5f));
//canvasSlot->SetAlignment(FVector2D(0.5f, 0.5f));
//canvasSlot->SetOffsets(FMargin(0, 0, 10, 10));
//RootWidget->Slot = canvasSlot;
UCanvasPanelSlot* RootWidgetSlot = Cast<UCanvasPanelSlot>(RootWidget->Slot);
if (RootWidgetSlot)
{
RootWidgetSlot->SetAnchors(FAnchors(0.f, 0.f, 1.f, 1.f));
RootWidgetSlot->SetOffsets(FMargin(0.f, 0.f));
}
else
{
//RootWidgetSlot->SetAnchors(FAnchors(0.f, 0.f, 1.f, 1.f));
//RootWidgetSlot->SetOffsets(FMargin(0.f, 0.f));
//RootWidget->Slot = RootWidgetSlot;
}
WidgetTree->RootWidget = RootWidget;
}
WidgetTree->Modify();
if (RootWidget && WidgetTree)
{
testTxtBlock = WidgetTree->ConstructWidget<UTextBlock>(UTextBlock::StaticClass(), FName(TEXT("testTxtBlock")));
RootWidget->AddChild(testTxtBlock);
uint64 MyValue = UINT64_MAX;
testTxtBlock->SetText(FText::FromString(FString::Printf(TEXT("%llu"),MyValue)));
UCanvasPanelSlot * textBoxSlot = Cast<UCanvasPanelSlot>(testTxtBlock->Slot);
if (textBoxSlot)
{
textBoxSlot->SetAutoSize(true);
}
editTxt = WidgetTree->ConstructWidget<UEditableText>(UEditableText::StaticClass(), FName(TEXT("editTxt")));
RootWidget->AddChild(editTxt);
//if (editTxt)
//{
// editTxt->SetText(FText("sss"));
//}
auto MyButton = WidgetTree->ConstructWidget<UButton>(UButton::StaticClass());
RootWidget->AddChild(MyButton);
auto MyButton2 = NewObject<UButton>(this);
RootWidget->AddChild(MyButton2);
}
PostEditChange();
#if WITH_EDITOR
//NewWisget
UFactory *factory = NewObject<UWidgetBlueprintFactory>();
char * name = "/Game/W_MyWidgetBlueprint";
UPackage *outer = CreatePackage(nullptr, UTF8_TO_TCHAR(name));
UClass *u_class = factory->GetSupportedClass();
char *obj_name = strrchr(name, '/') + 1;
if (u_class->IsChildOf<UBlueprint>())
{
if (FindObject<UBlueprint>(outer, UTF8_TO_TCHAR(obj_name)))
{
}
}
UObject *u_object = factory->FactoryCreateNew(u_class, outer, FName(UTF8_TO_TCHAR(obj_name)), RF_Public | RF_Standalone, nullptr, GWarn);
FAssetRegistryModule::AssetCreated(u_object);
outer->MarkPackageDirty();
UWidgetBlueprint* widget = Cast<UWidgetBlueprint>(u_object);
widget->Modify();
UCanvasPanel* slot1 = widget->WidgetTree->ConstructWidget<UCanvasPanel>(UCanvasPanel::StaticClass(),FName(TEXT("Slot1")));
UImage* image1 = widget->WidgetTree->ConstructWidget<UImage>(UImage::StaticClass(),
FName(TEXT("image1")));
slot1->AddChild(image1);
//UCanvasPanelSlot* slot2 = widget->WidgetTree->ConstructWidget<UCanvasPanelSlot>(UCanvasPanelSlot::StaticClass(),
// FName(TEXT("Slot1")));
UTextBlock* text_block = widget->WidgetTree->ConstructWidget<UTextBlock>(UTextBlock::StaticClass(),
FName(TEXT("text_block")));
text_block->Text = FText::FromString(FString("Hellow"));
//slot2->Content = text_block;
UPanelWidget* rootWidgetTemp = Cast<UPanelWidget>(widget->WidgetTree->RootWidget);
rootWidgetTemp->ClearChildren();
rootWidgetTemp->AddChild(slot1);
rootWidgetTemp->AddChild(image1);
widget->PostEditChange();
#endif
}
TSharedRef<SWidget> UAutoUIWidget::RebuildWidget()
{
InitTest();
TSharedRef<SWidget> widget = Super::RebuildWidget();
return widget;
}
<file_sep>/AutoUI/Source/AutoUI/MyUserWidget.h
// Fill out your copyright notice in the Description page of Project Settings.
#pragma once
#include "CoreMinimal.h"
#include "Blueprint/UserWidget.h"
#include "Runtime/UMG/Public/UMG.h"
#include "MyUserWidget.generated.h"
/**
*
*/
UCLASS()
class AUTOUI_API UMyUserWidget : public UUserWidget
{
GENERATED_BODY()
//public:
// UPROPERTY(meta = (BindWidgetOptional))
// class UEditableTextBox* HealthProgressBar;
// UPROPERTY(VisibleAnywhere, BlueprintReadOnly, Category = "State",meta=(BindWidget))
// float Energy;
//
// void NativeConstruct() override;
// UPROPERTY(EditDefaultsOnly, BlueprintReadOnly, Category = "State")
//
// float Energy2;
//
//private:
// UPROPERTY(VisibleAnywhere, BlueprintReadWrite, Category = "Power", meta = (AllowPrivateAccess = true))
// float PowerLevel;
//
virtual void OnCreationFromPalette() override;
};
<file_sep>/AutoUI/Plugins/AutoGenerateUMGByXML/Source/AutoGenerateUMGByXML/Public/AutoGenerateUMGByXMLCommands.h
// Copyright 1998-2018 Epic Games, Inc. All Rights Reserved.
#pragma once
#include "CoreMinimal.h"
#include "Framework/Commands/Commands.h"
#include "AutoGenerateUMGByXMLStyle.h"
class FAutoGenerateUMGByXMLCommands : public TCommands<FAutoGenerateUMGByXMLCommands>
{
public:
FAutoGenerateUMGByXMLCommands()
: TCommands<FAutoGenerateUMGByXMLCommands>(TEXT("AutoGenerateUMGByXML"), NSLOCTEXT("Contexts", "AutoGenerateUMGByXML", "AutoGenerateUMGByXML Plugin"), NAME_None, FAutoGenerateUMGByXMLStyle::GetStyleSetName())
{
}
// TCommands<> interface
virtual void RegisterCommands() override;
public:
TSharedPtr< FUICommandInfo > PluginAction;
};
| 7232a93885fb9966b32451478ff746bae85b17cb | [
"Markdown",
"C++"
] | 11 | C++ | bianchengxiaosheng/Unreal4-Dynami-Umg-Test | 4088b914f3be43cfbe7b5c185733c91bd06b4679 | 2ce5efce7dbbb68bffc96e4f5f15186b1e166743 | |
refs/heads/master | <file_sep>define(function(){
var isType = function( type ){
return function( obj ){
return Object.prototype.toString.call( obj ) === "[object " + type + "]";
};
}
var type = {};
type.isNum = isType( "Number" );
type.isString = isType( "String" );
type.isObject = isType( "Object" );
type.isFunc = isType( "Function" );
type.isArray = Array.isArray || isType( "Array" );
return type;
});<file_sep>// 下面的代码是Breath的底层模块
// 主要是用来处理Dom操作、CSS操作、JavaScript事件处理、class操作、data操作、动画操作
(function(window, document){
var guid = 1;
var Breath = window.Breath = function( selector, context ){
return new Breath.fn.init( selector, context || document);
};
// Breath的缓存系统
Breath.cache = {
__event : {}, // 这个是用来缓存用户的事件的
__data : {}, // 这个是用来缓存用户保存的数据的
__animation : {} // 这个是用来缓存用户设置的动画的
};
Breath.regExp = {
isTag: /^<[\w\W]+>$/, //这个正则表达式是用来检测完整的标签的
};
// 下面的代码是Breath的类型判断系统
Breath.type = function( obj ){
return /^\[object (\w+)\]$/.exec( Object.prototype.toString.call( obj ) )[1].toLowerCase();
};
Breath.isType = function( type ){
return function( obj ){
return Breath.type( obj ) === type;
}
};
Breath.isArray = Array.isArray || Breath.isType('array');
Breath.isString = Breath.isType('string');
Breath.isFunction = Breath.isType('function');
Breath.isObject = Breath.isType('object');
Breath.isRegExp = Breath.isType('regexp');
Breath.isNumber = Breath.isType('number');
Breath.isNaN = window.isNaN;
Breath.isDom = function( el ){
return !!( typeof el === 'object' && el.nodeType && ( el.nodeType === 1 || el.nodeType == 9 ) );
};
Breath.isWindow = function( el ){
var type = Breath.type( el );
return type === 'window' || type === 'global' || type === 'domwindow';
};
Breath.isNodeList = function( el ){
return Breath.type( el ) === 'nodelist';
};
// 判断对象是否为Breath对象
Breath.isBreath = function( el ){
return el instanceof Breath.prototype.init;
};
var __proto__ = Breath.fn = Breath.prototype = [];
// 下面的代码是用来进行扩展的
Breath.extend = Breath.prototype.extend = function(){
var deep = false;
var target = arguments[0];
var i = 1;
if( arguments.length <= 1 && !Breath.isObject( arguments[0] ) && !Breath.isFunction( arguments[0] ) ){
return this;
}
if( arguments.length <= 1 ){
target = this;
--i;
}
if( Breath.type( arguments[ 0 ] ) === 'boolean' ){
deep = arguments[0];
target = arguments[i];
++i;
}
for(; i < arguments.length; ++i){
if( deep ){
for( var key in arguments[i] ){
if( Breath.isObject( arguments[i][key] ) || Breath.isArray( arguments[i][key] ) ){
var tempTarget = Breath.isArray( arguments[i][key] ) ? [] : {};
target[key] = arguments.callee( true, tempTarget, arguments[i][key] );
}else{
target[key] = arguments[i][key];
}
}
}else{
for( var key in arguments[i] ){
target[key] = arguments[i][key];
}
}
}
return target;
};
// 下面的代码是用来对Breath进行扩展的
Breath.extend({
each: function(el, fn){
for(var i = 0; i < el.length; ++i){
fn.call( el[i], i, el[i], el );
}
},
toArray: function( obj ){
return Array.prototype.slice.call( obj );
},
eventSupport: function( eventType ){
eventType = "on" + eventType;
// DOM0事件检测
var el = document.createElement('breath');
var isSupport = eventType in el;
if( !isSupport && el.setAttribute && el.getAttribute ){
el.setAttribute( eventType, '' );
isSupport = Breath.isFunction( el.getAttribute( eventType ) );
}
if( isSupport ){
return true;
}
eventType = eventType.replace(/^on/, '');
// fullscreeneventchange事件检测
// if( eventType === 'fullscreeneventchange' ){
// var pfx = [ '', 'webkit', 'moz', 'o', 'ms' ];
// for( var i = 0; i < pfx.length; ++i ){
// if( Breath.isFunction( document[ pfx[i] + 'CancelFullScreen' ] ) && document[ pfx[i] + 'FullscreenEnabled' ] === true ){
// return true;
// }
// }
// // 对于IE下的fullscreeneventchange事件检测
// if( Breath.isFunction( document['msExitFullscreen' ] ) && document[ 'msFullscreenEnabled' ] === true ){
// return true;
// }
// // transitionEnd事件检测
// }else if( eventType === 'transitionEnd' ){
// isSupport = {
// isSupport: false
// };
// var obj = {
// TransitionEvent : 'transitionend' ,
// WebkitTransitionEvent : 'webkitTransitionEnd' ,
// OTransitionEvent : 'oTransitionEnd' ,
// oTransitionEvent : 'otransitionEnd'
// };
// for(var name in obj){
// if( window[name] ){
// isSupport.delegate = obj[name];
// break;
// }
// try{
// document.createEvent(name);
// isSupport.delegate = obj[name];
// break;
// }catch(ex){}
// }
// return isSupport;
// }
// // DOMMouseScroll事件检测
// isSupport = {
// mousewheel: {
// isSupport: false ,
// delegate: 'DOMMouseScroll'
// },
// DOMContentLoaded: true
// }[ eventType ] || false;
// return isSupport;
},
noop: function(){
}
});
// 下面的代码是用来进行对dom操作进行扩展的
Breath.extend( true, __proto__, {
init: function(){
if( !arguments[0] ){
return this;
}
// 下面的代码是用来处理传过来的对象是否为Breath对象
if( Breath.isBreath( arguments[0] ) ){
return arguments[0];
}
// 下面的代码是用来处理传来的参数是function的情况
if( Breath.isFunction( arguments[0] ) ){
return this.ready( arguments[0] );
}
// 选择或创建指定的元素
if( Breath.isString( arguments[0] ) ){
var selector = arguments[0].trim();
var context = null;
if( arguments[1] && ( Breath.isDom( arguments[1] ) || Breath.isString( arguments[1] ) || Breath.isBreath( arguments[1] ) ) ){
context = Breath( arguments[1] );
}
context = context && context[0];
context = context || document ;
var elements = this[ Breath.regExp.isTag.test( selector ) ? "createElement" : "query"]( selector, context );
for( var i = 0; i < elements.length; ++i ){
this.push( elements[i] );
}
return this;
}
// 下面的代码是用来处理传来的是数组的情况
if( Breath.isArray( arguments[0] ) || Breath.isNodeList( arguments[0] ) ){
for( var i = 0; i < arguments[0].length; ++i ){
this.push( arguments[0][i] );
}
return this;
}
this.push( arguments[0] );
return this;
},
createElement: function( elementString ){
var docTemp = document.implementation.createHTMLDocument( null );;
docTemp.body.innerHTML = elementString;
return docTemp.body.childNodes;
},
query: function( selector, context ){
return context.querySelectorAll( selector );
},
eq: function( index ){
return Breath( this[index] );
},
append: function( selector ){
this.insert( this, selector, 'beforeend' );
return this;
},
appendTo: function( selector ){
this.insert( selector, this, 'beforeend' );
return this;
},
prepend: function( selector ){
this.insert( this, selector, 'afterbegin' );
return this;
},
prependTo: function( selector ){
this.insert( selector, this, 'afterbegin' );
return this;
},
insertBefore: function( selector ){
this.insert( this, selector, 'beforebegin' );
return this;
},
insertAfter: function( selector ){
this.insert( this, selector, 'afterend' );
return this;
},
insert: function( targetEl, orignEl, position ){
// 对于Breath对象来说,我们是需要保留其先前注册的事件和数据的,所以这里我们不采用优化的方式去做
if( Breath.isBreath( orignEl ) ){
Breath( targetEl ).each(function( index, targetTmp ){
for( var i = orignEl.length - 1; i >= 0; --i ){
targetTmp.insertAdjacentElement || ( targetTmp.insertAdjacentElement = insertAdjacentElement );
targetTmp.insertAdjacentElement( position, orignEl[ i ] );
}
});
// 对于非Breath对象,我们采用,documentFragment去做,从而优化整个这个框架的性能
}else{
var docFragment = document.createDocumentFragment();
Breath( orignEl ).each(function( index, elTemp ){
docFragment.appendChild( elTemp );
});
var wraper = Breath('<div></div>')[0];
wraper.appendChild( docFragment );
var html = wraper.innerHTML;
Breath( targetEl ).each(function( index, elTemp ){
elTemp.insertAdjacentHTML( position, html );
});
}
return this;
function insertAdjacentElement( where, node ){
switch (where) {
case "beforebegin":
this.parentNode.insertBefore( node, this );
break;
case "afterbegin":
this.insertBefore( node, this.firstChild );
break;
case "beforeend":
this.appendChild( node );
break;
case "afterend":
if ( this.nextSibling ){
this.parentNode.insertBefore(node, this.nextSibling);
}else{
this.parentNode.appendChild(node);
}
break;
}
}
},
find: function( selector ){
var result = Breath();
if( Breath.isString( selector ) && !( Breath.regExp.isTag.test( selector ) ) ){
this.each(function(index, elTemp){
Breath( selector, elTemp ).each(function(index, temp ){
result.push( temp );
});
});
}
return result;
},
children: function( selector ){
var result = Breath();
if( Breath.isString( selector ) && !( Breath.regExp.isTag.test( selector ) ) ){
this.each(function(index, parentElement){
Breath( selector, parentElement ).each(function(index, element ){
element.parentNode === parentElement && result.push( element );
});
});
}
return result;
},
parent: function(){
if( this.length ){
return Breath( this[0].parentNode );
}
return null;
},
remove: function(){
this.each(function(index, elTemp){
elTemp.parentNode.removeChild( elTemp );
});
return this;
},
empty: function(){
this.each(function( index, el ){
el.innerHTML = '';
});
return this;
},
each: function( fn ){
Breath.each( this, fn );
return this;
},
clone: function(){
if( this.length ){
return Breath( this[0].outerHTML ) ;
}
return null;
},
count: function(){
return this.length;
}
});
// 下面的代码是用来对元素的class进行操作的
Breath.extend( true, __proto__, {
addClass: function( className ){
if( !Breath.isString( className ) ){
className = '';
}
className = className.trim().split(' ');
this.each(function( index, elTemp ){
var classOfElement = ' ' + elTemp.className.trim() + ' ';
for(var i = 0; i < className.length; ++i ){
var temp = className[i].trim();
if( !!temp && classOfElement.indexOf( ' ' + temp + ' ' ) < 0 ){
classOfElement += temp + ' ';
}
}
elTemp.className = classOfElement.trim();
});
return this;
},
removeClass: function( className ){
if( !Breath.isString( className ) ){
className = '';
}
className = className.trim().split(' ');
this.each(function( index, elTemp ){
var classOfElement = ' ' + elTemp.className.trim() + ' ';
for(var i = 0; i < className.length; ++i ){
var temp = className[i].trim();
temp && ( classOfElement = classOfElement.replace( ' ' + temp + ' ', ' ' ) );
}
elTemp.className = classOfElement.trim();
});
return this;
},
toggleClass: function( className ){
if( !Breath.isString( className ) ){
className = '';
}
className = className.trim().split(' ');
this.each(function( index, elTemp ){
var classOfElement = ' ' + elTemp.className.trim() + ' ';
var copy = ' ' + elTemp.className.trim() + ' ';
for(var i = 0; i < className.length; ++i ){
var temp = className[i].trim();
if( temp ){
// 下面的代码是用来增加class
if( copy.indexOf( ' ' + temp + ' ' ) < 0 && classOfElement.indexOf( ' ' + temp + ' ' ) < 0 ){
classOfElement += temp + ' ';
// 下面的代码是用来删除class
}else if( copy.indexOf( ' ' + temp + ' ' ) >= 0 && classOfElement.indexOf( ' ' + temp + ' ' ) >= 0 ){
classOfElement = classOfElement.replace( ' ' + temp + ' ', ' ' );
}
}
}
elTemp.className = classOfElement.trim();
});
return this;
},
hasClass: function( className ){
if( !this.length || !Breath.isString( className ) || !className.trim() ){
return false;
}
var classOfElement = ' ' + this[0].className + ' ';
className = className.trim().split(' ');
for( var i = 0; i < className.length; ++i ){
if( classOfElement.indexOf( ' ' + className[i] + ' ' ) < 0 ){
return false;
}
}
return true;
}
});
// 下面的代码是用来对event模块进行封装的
(function(){
function handleEvent( evt ){
var returnValue = true;
evt = fixEvent( evt );
var eventId = Breath.isWindow( this ) ? ( this.windowData || {} )['eventId'] : this.eventId;
var handlers = ( Breath.cache.__event[ eventId ] || {} )[ evt.type ] || {};
for( var i in handlers ){
if( handlers[i].call(this, evt ) === false ){
returnValue = false;
}
}
return returnValue;
}
function fixEvent( evt ){
var evt = evt || window.event;
evt.stopPropagation = evt.stopPropagation || fixEvent.stopPropagation;
evt.preventDefault = evt.preventDefault || fixEvent.preventDefault ;
// 下面的代码是用来兼容firefox的wheelscroll事件
if( evt.type === 'DOMMouseScroll ' ){
evt.wheelDelta = evt.detail * - 40;
}
return evt;
}
fixEvent.stopPropagation = function(){
this.cancelBubble = true;
};
fixEvent.preventDefault = function(){
this.returnValue = false;
};
Breath.extend( true, __proto__, {
on: function( eventType, fn ){
this.each(function( index, el ){
var eventId;
if( Breath.isWindow( el ) ){
el.windowData || ( el.windowData = {} );
eventId = el.windowData.eventId;
}else{
eventId = el.eventId;
}
if( !eventId ){
eventId = guid++;
Breath.isWindow( el ) ? ( el.windowData.eventId = eventId ) : el.eventId = eventId;
Breath.cache.__event[ eventId ] = {};
}
fn.eventId = guid++;
var cache = Breath.cache.__event[ eventId ][ eventType ];
// 下面的代码是用来检测当前元素是否已经设置过该类型的事件了,如果没有的话,设置缓存保存事件回调函数,并且绑定对应的事件
if( !cache ){
cache = {};
// 这里的函数只能够添加一次,因为每添加一次,函数都会被调用一次
el.addEventListener( eventType, handleEvent, false );
}
cache[ fn.eventId ] = fn;
Breath.cache.__event[ eventId ][ eventType ] = cache;
});
return this;
},
// 对于事件的绑定off是有问题的,请在后面的进行修改
off: function( eventType, fn ){
this.each(function( index, el ){
var eventId = Breath.isWindow( el ) ? ( el.windowData && el.windowData.eventId ) : el.eventId;
var cache = Breath.cache.__event[ eventId ] || {};
if( fn === undefined ){
delete cache[ eventType ];
} else {
if( cache[ eventType ] ){
delete cache[ eventType ][ fn.eventId ];
for( var i in cache[ eventType ]){};
if( i === undefined ){
delete cache[ eventType ];
}
}
}
// 当该事件的没有对应的处理函数的时候,我们删除该元素所绑定的函数
if( cache[ eventType ] === undefined ){
el.removeEventListener( eventType, handleEvent, false );
}
for( var i in cache ){};
if( i === undefined ){
delete Breath.cache.__event[ el.eventId ];
delete ( Breath.isWindow( el ) ? ( Breath.windowData || {} ) : el )["eventId"];
}
});
return this;
},
ready: function( fn ){
return Breath(document).on( "DOMContentLoaded", fn );
},
transitionEnd: function( callback ){
this.on( transitionEndEventName(), callback );
return this;
},
offTransitonEnd: function( callback ){
this.off( transitionEndEventName(), callback );
return this;
},
mouseWheel: function( callback ){
this.on( mouseWheelEventName(), callback );
return this;
},
offMouseWheel: function( callback ){
this.off( mouseWheelEventName(), callback );
return this;
},
fullScreenChange: function( callback ){
var names = [
'fullscreenchange' ,
'webkitfullscreenchange' ,
'mozfullscreenchange' ,
'MSFullscreenChange'
];
for( var i = 0; i < names.length; ++i ){
Breath( document ).on( names[i], callback );
}
return this;
},
offFullScreenChange: function( callback ){
var names = [
'fullscreenchange' ,
'webkitfullscreenchange' ,
'mozfullscreenchange' ,
'MSFullscreenChange'
];
for( var i = 0; i < names.length; ++i ){
Breath( document ).off( names[i], callback );
}
return this;
},
keyup: function( callback ){
this.each(function( index, elTmp ){
elTmp.tabIndex = -1;
});
this.on( 'keyup', callback );
return this;
},
offKeyup: function( callback ){
this.each(function( index, elTmp ){
elTmp.removeAttribute('tabIndex');
});
this.off( 'keyup', callback );
return this;
},
// 下面的代码是用来实现事件代理的,由于对于实现的框架而言这个功能暂且不需要,所以这个功能后期实现
delegate: function( selector, eventType, fn ){
return this;
},
trigger: function( eventType, data ){
var evt = document.createEvent('CustomEvent');
evt.initCustomEvent( eventType, true, true, data || null );
this.each( function(index, elTemp){
elTemp.dispatchEvent( evt );
});
return this;
}
});
// 下面的函数是用来获取mousewheel的事件名的
function mouseWheelEventName(){
if( mouseWheelEventName.eventName ){
return mouseWheelEventName.eventName;
}
try{
document.createEvent('MouseScrollEvents');
mouseWheelEventName.eventName = 'DOMMouseScroll';
}catch(ex){
mouseWheelEventName.eventName = 'mousewheel';
}
return mouseWheelEventName.eventName;
}
// 下面的函数是用来获取transitionEnd事件的名称的
function transitionEndEventName(){
// 惰性检测事件的名称,从而提高代码的效率
if( transitionEndEventName.eventName ){
return transitionEndEventName.eventName;
}
var eventName;
var obj = {
TransitionEvent : 'transitionend' ,
WebkitTransitionEvent : 'webkitTransitionEnd' ,
OTransitionEvent : 'oTransitionEnd' ,
oTransitionEvent : 'otransitionEnd'
};
for( var key in obj ){
if( window[ key ] ){
transitionEndEventName.eventName = obj[ key ];
break;
}
try{
document.createEvent( key );
transitionEndEventName.eventName = obj[ key ];
break;
}catch(ex){}
}
return transitionEndEventName.eventName;
}
})();
// 下面的代码是用来处理元素的css模块
Breath.extend( true, __proto__, {
css: function(){
if( !( Breath.isString( arguments[0] ) || Breath.isObject( arguments[0] ) ) ){
return this;
}
// get style
if( Breath.isString( arguments[0] ) && arguments[1] === undefined ){
return this.getStyle( arguments[0] );
}
//set style
var style = null;
if( Breath.isObject( arguments[0] ) ){
style = arguments[0];
}else if( Breath.isString( arguments[0] ) && ( Breath.isString( arguments[1] ) || arguments[1] === null ) ){
style = {};
style[ arguments[0] ] = arguments[1];
}
if( style ){
this.setStyle( style );
}
return this;
},
show: function(){
this.css('display', 'block');
return this;
},
hide: function(){
this.css('display', 'none');
return this;
},
width: function(){
if( arguments[0] === undefined ){
return parseFloat( $(this[0]).css('width') );
}
var width = parseFloat( arguments[0] );
if( !Breath.isNaN( width ) ){
this.css( 'width', width + 'px' );
}
return this;
},
height: function(){
if( arguments[0] === undefined ){
return parseFloat( $(this[0]).css('height') );
}
var height = parseFloat( arguments[0] );
if( !isNaN( height ) ){
this.css( 'height', height + 'px' );
}
return this;
},
top: function(){
if( arguments[0] === undefined ){
var result = $(this[0]).css('top');
result = result === 'auto' ? 0 : parseFloat( result ) ;
return result;
}
var top = parseFloat( arguments[0] );
if( !isNaN( top ) ){
this.css( 'top', top + 'px' );
}
return this;
},
left: function(){
var left;
if( arguments[0] === undefined ){
left = $(this[0]).css('top');
left = left === 'auto' ? 0 : parseFloat( left ) ;
return left;
}
left = parseFloat( arguments[0] );
if( !isNaN( left ) ){
this.css( 'left', left + 'px' );
}
return this;
},
getStyle: function( styleName ){
if( this.length == 0 ){
return null;
}
styleName = this.fixedStyleName( styleName );
return window.getComputedStyle( this[0], null )[ styleName ] || null;
},
setStyle: function( style ){
var self = this;
this.each(function( index, elTemp ){
for(var key in style){
var styleName = self.fixedStyleName( key );
if( styleName ){
elTemp.style[ styleName ] = style[ key ];
}
}
});
},
fixedStyleName: function( styleName ){
// 下面的代码是用来将css的名字转化成驼峰模式
styleName.replace( /-(\w)/g , function(all, letter){
return letter.toUpperCase();
});
var breath = document.createElement( 'breath' );
var style = window.getComputedStyle( breath, null );
// 下面的代码是用来兼容float的
var floatName = ['styleFloat', 'cssFloat', 'float'];
if( floatName.indexOf( styleName ) >= 0 ){
for(var i = 0; i < floatName.length; ++i ){
if( floatName[i] in style ){
return floatName[i];
}
}
}
// 检测不带前缀的样式名是否支持
var tempName = styleName[0] + styleName.substr(1);
if( tempName in breath.style ){
return tempName;
}
// 检测带前缀的样式是否支持
var pfx = [ 'webkit', 'moz', 'ms', 'o' ];
for(var i = 0; i < pfx.length; ++i){
var tempName = pfx[i] + styleName;
if( tempName in style ){
return tempName;
}
}
// 无法识别的样式我们返回null
return null;
}
});
// 下面的代码是用来处理Breath的data模块的(包括html5中的以data开头自定义的数据)
(function($, __proto__){
$.extend( true, __proto__ , {
data: function(){
// get data
if( arguments[0] === undefined || ( $.isString( arguments[0] ) && arguments[1] === undefined ) ){
return getData.call( this[0], this[0], arguments[0] );
}
// set data
if( $.isObject( arguments[0] ) || ( $.isString( arguments[0] ) && arguments[1] !== undefined ) ){
var tmpData = null;
if( $.isString( arguments[0] ) ){
tmpData = {};
tmpData[ arguments[0] ] = arguments[1];
}
tmpData || ( tmpData = arguments[0] );
this.each(function( index, elTmp ){
setData.call( elTmp, elTmp, tmpData );
});
}
return this;
},
removeData: function(){
},
// 用来设置、修改或删除key为name的值,如果value为null的话,表明删除对应的数据
dataset: function( name, value ){
if( this.length && $.isString( name ) && name !== "" ){
// 获取第一个元素的数据
if( value === undefined ){
value = this[0].dataset[ name ];
value = value === undefined ? null : value;
// 通过正则表达式来判断当前的数据是否是object或array的json格式
if( /^\{[\s\S]*\}$|^\[[\s\S]*\]$/.test( value ) ){
value = JSON.parse( value );
}
return value;
// 删除每个元素的数据
}else if( value === null ){
this.each(function( index, elTmp ){
delete elTmp.dataset[ name ];
});
// 为每个元素添加或修改对应的数据
}else{
value = $.isObject( value ) || $.isArray( value ) ? JSON.stringify( value ) : value;
this.each(function( index, elTmp ){
elTmp.dataset[ name ] = value;
});
}
}
return this;
},
});
function setData(el, data){
var dataId = null;
// 防止污染全局作用域
if( $.isWindow( el ) ){
el.windowData || ( el.windowData = {} );
dataId = el.windowData['dataId'];
}else{
dataId = el['dataId'];
}
if( !dataId ){
$.isWindow( el ) ? ( el.windowData['dataId'] = guid ) : ( el['dataId'] = guid );
dataId = guid++;
}
var cache = $.cache.__data[dataId] || {};
for( var key in data ){
cache[key] = data[key];
}
$.cache.__data[dataId] = cache;
}
function getData(el, name){
var dataId = $.isWindow( el ) ? ( window.windowData || {} )['dataId'] : el.dataId;
var cache = $.cache.__data[ dataId ];
// 获取元素el所有的存储的数据
if( !name ){
return cache || null;
}
// 获取制定名称的数据
var data = ( cache || {} )[ name ];
return data === undefined ? null : data;
}
})( Breath, __proto__ );
// 下面的代码使用处理Breath的属性模块的
Breath.extend( true, __proto__, {
});
// 下面的代码是用来使元素全屏的
Breath.extend( true, __proto__, {
// 对于IE11的全屏的话必须通过用户启动的事件(如按钮事件)调用msRequestFullscreen才有效,不然没有任何效果
fullScreen: function(){
if( this[0] ){
var pfx = [ '', 'webkit', 'moz', 'ms' ];
for( var i = 0; i < pfx.length; ++i ){
// chrome firefox
var funcName = pfx[i] + 'RequestFullScreen';
funcName = funcName[0].toLowerCase() + funcName.substr(1);
if( funcName in this[0] ){
this[0][ funcName ]();
break;
}
// w3c
var funcName = pfx[i] + 'RequestFullscreen';
funcName = funcName[0].toLowerCase() + funcName.substr(1);
if( funcName in this[0] ){
this[0][ funcName ]();
break;
}
}
}
return this;
},
cancelFullScreen: function(){
var pfx = [ '', 'webkit', 'moz', 'ms', 'moz' ];
for( var i = 0; i < pfx.length; ++i ){
// chrome firefox
var funcName = pfx[i] + 'CancelFullScreen';
funcName = funcName[0].toLowerCase() + funcName.substr(1);
if( funcName in document ){
document[ funcName ]();
break;
}
// w3c
var funcName = pfx[i] + 'ExitFullscreen';
funcName = funcName[0].toLowerCase() + funcName.substr(1);
if( funcName in document ){
document[ funcName ]();
break;
}
}
return this;
},
// 下面的函数是用来判断当前元素是否全屏的
isFullScreen: function(){
var names = [
'fullscreenElement' ,
'webkitFullscreenElement' ,
'mozFullScreenElement' ,
'msFullscreenElement'
];
for( var i = 0; i < names.length; ++i ){
if( document[ names[i] ] === this[0] ){
return true;
}
}
return false;
}
});
// animation model
(function($, __proto__){
// 下面的代码是JavaScript的缓动公式
var CSSCubicBezier = {
'in': 'ease-in' ,
'out': 'ease-out' ,
'in-out': 'ease-in-out' ,
'snap': 'cubic-bezier(0,1,.5,1)' ,
'linear': 'cubic-bezier(0.250, 0.250, 0.750, 0.750)' ,
'ease-in-quad': 'cubic-bezier(0.550, 0.085, 0.680, 0.530)' ,
'ease-in-cubic': 'cubic-bezier(0.550, 0.055, 0.675, 0.190)' ,
'ease-in-quart': 'cubic-bezier(0.895, 0.030, 0.685, 0.220)' ,
'ease-in-quint': 'cubic-bezier(0.755, 0.050, 0.855, 0.060)' ,
'ease-in-sine': 'cubic-bezier(0.470, 0.000, 0.745, 0.715)' ,
'ease-in-expo': 'cubic-bezier(0.950, 0.050, 0.795, 0.035)' ,
'ease-in-circ': 'cubic-bezier(0.600, 0.040, 0.980, 0.335)' ,
'ease-in-back': 'cubic-bezier(0.600, -0.280, 0.735, 0.045)' ,
'ease-out-quad': 'cubic-bezier(0.250, 0.460, 0.450, 0.940)' ,
'ease-out-cubic': 'cubic-bezier(0.215, 0.610, 0.355, 1.000)' ,
'ease-out-quart': 'cubic-bezier(0.165, 0.840, 0.440, 1.000)' ,
'ease-out-quint': 'cubic-bezier(0.230, 1.000, 0.320, 1.000)' ,
'ease-out-sine': 'cubic-bezier(0.390, 0.575, 0.565, 1.000)' ,
'ease-out-expo': 'cubic-bezier(0.190, 1.000, 0.220, 1.000)' ,
'ease-out-circ': 'cubic-bezier(0.075, 0.820, 0.165, 1.000)' ,
'ease-out-back': 'cubic-bezier(0.175, 0.885, 0.320, 1.275)' ,
'ease-out-quad': 'cubic-bezier(0.455, 0.030, 0.515, 0.955)' ,
'ease-out-cubic': 'cubic-bezier(0.645, 0.045, 0.355, 1.000)' ,
'ease-in-out-quart': 'cubic-bezier(0.770, 0.000, 0.175, 1.000)' ,
'ease-in-out-quint': 'cubic-bezier(0.860, 0.000, 0.070, 1.000)' ,
'ease-in-out-sine': 'cubic-bezier(0.445, 0.050, 0.550, 0.950)' ,
'ease-in-out-expo': 'cubic-bezier(1.000, 0.000, 0.000, 1.000)' ,
'ease-in-out-circ': 'cubic-bezier(0.785, 0.135, 0.150, 0.860)' ,
'ease-in-out-back': 'cubic-bezier(0.680, -0.550, 0.265, 1.550)'
};
$.extend( true, __proto__, {
animate: function(props, time, timingFunction, delay){
if( $.isObject( props ) ){
var options = {
time : 500 ,
delay: 0 ,
timingFunction: CSSCubicBezier[ 'linear' ]
};
time = parseInt( time );
$.isNaN( time ) || ( options.time = time );
delay = parseInt( delay );
$.isNaN( delay ) || ( options.delay = delay );
CSSCubicBezier[timingFunction] && ( options.timingFunction = CSSCubicBezier[timingFunction] );
// 下面的代码是用来将动画的数据缓存到队列中,从而使得动画能够顺序执行
var cache = $.cache.__animation;
this.each(function(index, elTmp){
var isWindow = $.isWindow( elTmp ) ;
var animateId = isWindow ? ( elTmp.windowData || {} )['animateId'] : elTmp.animateId ;
if( !animateId ){
if( isWindow ){
elTmp.windowData || ( elTmp.windowData = {} );
elTmp.windowData.animateId = guid;
}else{
elTmp.animateId = guid;
}
animateId = guid++;
}
var animateData = cache[ animateId ] || {};
// 缓存动画是否在进行中
animateData['animating'] === undefined && ( animateData['animating'] = false );
// 缓存动画的配置参数
$.isArray( animateData['options'] ) || ( animateData['options'] = [] );
animateData['options'].push( options );
// 缓存动画的属性
$.isArray( animateData['props'] ) || ( animateData['props'] = [] );
animateData['props'].push( props );
cache[ animateId ] = animateData;
// 如果没有开始动画的话,开始动画
if( !animateData['animating'] ){
animateData['animating'] = true;
var propsTmp = animateData['props'].shift();
var optionsTmp = animateData['options'].shift();
elTmp = $( elTmp );
elTmp.css( $.extend( true, {}, propsTmp, {
transition: 'all ' + optionsTmp.time + 'ms ' + optionsTmp.timingFunction + ' ' + optionsTmp.delay + 'ms'
}));
// 下面的事件名是需要改动的,请以后进行修改
var count = 0;
// elTmp.on('transitionEnd', function(){
// // 一个动画可能会涉及到多个属性,多个熟悉会触发多次transitionEnd事件,我们只处理最后一次触发
// if( Object.keys( propsTmp ).length < ++count ){
// return;
// }
// count = 0;
// if( !( animateData['props'].length && animateData['options'].length ) ){
// animateData['animating'] = false;
// elTmp.off( 'transitionEnd' );
// console.log( 'transition end' );
// return;
// }
// propsTmp = animateData['props'].shift();
// optionsTmp = animateData['options'].shift();
// // 触发队列中的下一个动画
// elTmp.css( $.extend( true, {}, propsTmp, {
// transition: 'all ' + optionsTmp.time + 'ms ' + optionsTmp.timingFunction + ' ' + optionsTmp.delay + 'ms'
// }));
// });
elTmp.transitionEnd(function(){
// 一个动画可能会涉及到多个属性,多个熟悉会触发多次transitionEnd事件,我们只处理最后一次触发
if( Object.keys( propsTmp ).length < ++count ){
return;
}
count = 0;
if( !( animateData['props'].length && animateData['options'].length ) ){
animateData['animating'] = false;
elTmp.offTransitonEnd();
console.log( 'transition end' );
return;
}
propsTmp = animateData['props'].shift();
optionsTmp = animateData['options'].shift();
// 触发队列中的下一个动画
elTmp.css( $.extend( true, {}, propsTmp, {
transition: 'all ' + optionsTmp.time + 'ms ' + optionsTmp.timingFunction + ' ' + optionsTmp.delay + 'ms'
}));
});
}
});
}
return this;
},
fadeIn: function(time, timingFunc, delay){
this.each(function( index, elTmp ){
$elTmp = $(elTmp);
if( $elTmp.css('opacity') == 1 ){
return;
}
$elTmp.animate( { opacity: 1 } , time, timingFunc, delay );
});
return this;
},
fadeOut: function(time, timingFunc, delay){
this.each(function( index, elTmp ){
$elTmp = $(elTmp);
if( $elTmp.css('opacity') == 0 ){
return;
}
$elTmp.animate( { opacity: 0 } , time, timingFunc, delay );
});
return this;
},
fadeTo: function( opacity, time, timingFunc, delay ){
opacity = parseFloat( opacity );
if( $.isNaN( opacity ) ){
return this;
}
opacity > 1 && ( opacity = 1 );
opacity < 0 && ( opacity = 0 );
this.each(function(index, elTmp){
$elTmp = $(elTmp);
if( $elTmp.css('opacity') == opacity ){
return;
}
$elTmp.animate( { opacity: opacity } , time, timingFunc, delay );
});
return this;
},
moveBy: function(offsetX, offsetY, time, timingFunc, delay){
offsetX = parseInt( offsetX );
offsetY = offsetY === undefined ? 0 : parseInt( offsetY );
if( $.isNaN( offsetX ) || $.isNaN( offsetY ) ){
return this;
}
this.each(function( index, elTmp ){
if( !( offsetX || offsetY ) ){
return;
}
var $elTmp = $(elTmp);
elTmp.position || ( elTmp.position = {} );
elTmp.position.top = elTmp.position.top ? elTmp.position.top + offsetY : $elTmp.top() + offsetY ;
elTmp.position.left = elTmp.position.left ? elTmp.position.left + offsetX : $elTmp.left() + offsetX ;
$elTmp.animate({
top : elTmp.position.top + 'px' ,
left : elTmp.position.left + 'px'
}, time, timingFunc, delay);
});
return this;
},
xBy: function(offsetX, time, timingFunc, delay){
this.moveBy( offsetX, 0, time, timingFunc, delay );
return this;
},
yBy: function(offsetY, time, timingFunc, delay){
this.moveBy( 0, offsetY, time, timingFunc, delay );
return this;
},
moveTo: function(posX, posY, time, timingFunc, delay){
posX = parseInt( posX );
posY = parseInt( posY );
if( $.isNaN( posX ) || $.isNaN( posY ) ){
return this;
}
this.each(function( index, elTmp ){
$elTmp = $(elTmp);
if( $elTmp.top() == posY && $elTmp.left() == posX ){
return;
}
elTmp.position = {
top : posY ,
left: posX
};
$elTmp.animate({
top : elTmp.position.top + 'px' ,
left: elTmp.position.left + 'px'
}, time, timingFunc, delay );
});
return this;
},
xTo: function(posX, time, timingFunc, delay){
posX = parseInt( posX );
if( $.isNaN( posX ) ){
return this;
}
this.each(function( index, elTmp ){
$elTmp = $(elTmp);
if( $elTmp.left() == posX ){
return;
}
elTmp.position = {
left: posX
};
$elTmp.animate({
left: elTmp.position.left + 'px'
}, time, timingFunc, delay );
});
return this;
},
yTo: function(posY, time, timingFunc, delay){
posY = parseInt( posY );
if( $.isNaN( posY ) ){
return this;
}
this.each(function( index, elTmp ){
$elTmp = $(elTmp);
if( $elTmp.top() == posY ){
return;
}
elTmp.position = {
top : posY
};
$elTmp.animate({
top : elTmp.position.top + 'px'
}, time, timingFunc, delay );
});
return this;
},
scaleBy: function(scaleX, scaleY, time, timingFunc, delay){
scaleX = parseInt( scaleX );
scaleY = scaleY === undefined ? scaleX : parseInt( scaleY );
if( $.isNaN( scaleX ) || $.isNaN( scaleY ) || scaleX <= 0 || scaleY <= 0 ){
return this;
}
this.each(function( index, elTmp ){
if( scaleX == 1 && scaleY == 1 ){
return;
}
elTmp.scale || ( elTmp.scale = {} );
elTmp.scale.scaleX = elTmp.scale.scaleX ? ( elTmp.scale.scaleX * scaleX ) : scaleX;
elTmp.scale.scaleY = elTmp.scale.scaleY ? ( elTmp.scale.scaleY * scaleY ) : scaleY;
$(elTmp).animate({
transform: 'scale(' + elTmp.scale.scaleX + ', ' + elTmp.scale.scaleY + ')'
}, time, timingFunc, delay);
});
return this;
},
scaleTo: function(scaleX, scaleY, time, timingFunc, delay){
scaleX = parseInt( scaleX );
scaleY = scaleY === undefined ? scaleX : parseInt( scaleY );
if( $.isNaN( scaleX ) || $.isNaN( scaleY ) || scaleX <= 0 || scaleY <= 0 ){
return this;
}
this.each(function( index, elTmp ){
if( ( !elTmp.scale && scaleX == 1 && scaleY == 1 ) || ( elTmp.scale && elTmp.scale.scaleX == scaleX && elTmp.scale.scaleY == scaleY ) ){
return;
}
elTmp.scale = {
scaleX: scaleX ,
scaleY: scaleY
};
$(elTmp).animate({
transform: 'scale(' + scaleX + ', ' + scaleY + ')'
}, time, timingFunc, delay);
});
return this;
},
rotateBy: function( offsetDegree, time, timingFunc, delay){
offsetDegree = parseInt( offsetDegree );
if( $.isNaN( offsetDegree ) ){
return this;
}
this.each(function( index, elTmp ){
elTmp.degree = ( elTmp.degree || 0 ) + offsetDegree;
$(elTmp).animate({
transform: 'rotate(' + elTmp.degree + 'deg)'
}, time, timingFunc, delay);
});
return this;
},
rotateTo: function(degree, time, timingFunc, delay){
degree = parseInt( degree );
if( $.isNaN( degree ) ){
return this;
}
this.each(function( index, elTmp ){
// 如果状态不发生变化返回,不做任何处理
if( !( elTmp.degree || degree ) || elTmp.degree == degree ){
return;
}
elTmp.degree = degree;
$(elTmp).animate({
transform: 'rotate(' + degree + 'deg)'
}, time, timingFunc, delay);
});
return this;
}
});
})(Breath, __proto__);
Breath.fn.init.prototype = Breath.fn;
})(window, document);
// 下面的代码是用来创建PPT的框架的
(function($){
var guid = 1;
//
// 下面的代码里存在一个bug,那个bug就是不知道为什么preview_version上进行的一些列操作都没有效果,请在后面进行修复
//
var PPT = function(){
this.each(function( index, elTmp ){
var id_PPT = guid++;
// 下面的代码是用来保存PPT的数据的
var data_PPT = PPT.data[ id_PPT ] = {
PPT_playing : null ,
PPT_preview : null ,
steps_playing : null , // PPT所有页的Breath对象
steps_preview : null ,
data_steps : [] , // 每一页PPT的数据
index_curt_step : 0 // 当前播放的页数
};
// 这个是用来进行播放的
var playing_version = data_PPT.PPT_playing = $( elTmp );
// 这个是用来进行预览的
var preview_version = playing_version.clone().addClass( 'preview' ).appendTo( playing_version.parent() );
// 保存每个PPT的id
[ playing_version, preview_version ].forEach( function( version, index ){
version.data( 'id_PPT', id_PPT );
});
var steps_playing = data_PPT.steps_playing = playing_version.find( '.step' );
var steps_preview = data_PPT.steps_preview = preview_version.find( '.step' );
[ steps_playing, steps_preview ].forEach( function( steps, index ){
steps.each( function( index, step ){
step = $( step );
// 设置每一页的背景色
var background = step.dataset( 'background' ) ||
playing_version.dataset( 'background' ) || // 这里通过preview_version来获得也是可以的
PPT.config.step.background;
step.css( 'background', background );
// 设置每一页的页数(页数是从0开始的)
step.data( 'pageIndex', index );
});
});
steps_preview.on( 'dblclick', function(){
steps_playing.hide();
var index_curt_step = data_PPT.index_curt_step = $(this).data( 'pageIndex' );
steps_playing.eq( index_curt_step ).show().css({
width : '100%' ,
height : '100%' ,
top : 0 ,
left : 0
});
// 下面的代码是用来初始化上一页的step的
if( index_curt_step > 0 ){
var index_step = index_curt_step - 1;
var step = steps_playing.eq( index_step );
var data_step = data_PPT.data_steps[ index_step ];
PPT.transition.in[ data_step[ 'transitionIn' ] ].init( step );
}
// 下面的代码是用来初始化下一页的step的
if( index_curt_step < steps_playing.count() - 1 ){
var index_step = index_curt_step + 1;
var step = steps_playing.eq( index_step );
var data_step = data_PPT.data_steps[ index_step ];
PPT.transition.in[ data_step[ 'transitionIn' ] ].init( step );
}
playing_version.fullScreen();
});
playing_version.addClass( 'playing' ).keyup( function( evt ){
switch( evt.keyCode ){
case 37: // 左键
case 38: // 上键
PPT.prev( id_PPT, data_PPT.index_curt_step );
break;
case 39: // 右键
case 40: // 下键
PPT.next( id_PPT, data_PPT.index_curt_step );
break;
}
}).fullScreenChange(function(){
console.log( "wanglei is cool and kang is wanglei best friend" );
if( playing_version.isFullScreen() ){
playing_version.show();
preview_version.hide();
}else{
playing_version.hide();
preview_version.show();
}
});
playing_version.find('.step').each( function( index, step ){
step = $( '.step' );
var data = {
transitionIn : step.dataset('transitionIn') || 'default' ,
transitionOut : step.dataset('transitionOut') || 'default' ,
transitionInStart : step.dataset('transitionInStart') || $.noop ,
transitionInEnd : step.dataset('transitionInEnd') || $.noop ,
transitionOutStart: step.dataset('transitionOutStart') || $.noop ,
transitionOutEnd : step.dataset('transitionOutEnd') || $.noop
};
PPT.data[ id_PPT ]['data_steps'].push( data );
});
// 开始的时候预览版本显示,播放版本隐藏
playing_version.hide();
preview_version.show();
});
};
PPT.config = {
version : "0.0.1" , // 版本号
name : "Breath" , // 名称
step : {
background : "transparent" // 每一页的背景颜色
}
};
// 下面的代码是用来保存PPT中每一页的数据的
PPT.data = [];
// 下面的代码是用来扩展每一页PPT进入或出去的动画效果的
PPT.transition = {
in : {},
out: {}
};
PPT.transition.in.default = function( step ){
$(step).show();
};
PPT.transition.in.default.init = function( step ){
$(step).hide();
};
PPT.transition.out.default = function( step ){
$(step).hide();
};
// index表示当前播放的页面的页数
PPT.prev = function( id_PPT, index ){
if( index == 0 ){
return;
}
var data_PPT = PPT.data[ id_PPT ];
var steps = data_PPT['steps_playing'];
PPT.go( id_PPT, index - 1 );
};
// index表示当前播放的页面的页数
PPT.next = function( id_PPT, index ){
var data_PPT = PPT.data[ id_PPT ];
var steps = data_PPT['steps_playing'];
if( index == steps.count() -1 ){
return;
}
PPT.go( id_PPT, index + 1 );
};
// index表示将要播放的页面的页数
PPT.go = function( id_PPT, index ){
var data_PPT = PPT.data[ id_PPT ];
var steps = data_PPT['steps_playing'];
if( index < 0 || index >= steps.count() ){
return;
}
steps.hide();
data_PPT.index_curt_step = index;
steps.eq( index ).show();
};
$.fn.extend({
PPT: PPT
});
})(Breath);
window.$ = Breath;
$('.breath').PPT();
<file_sep>define( ["type"], function( type ){
var EventEmitter = {
cache: {}
};
EventEmitter.on = function( type, func ){
if( !(type && func) ){
console.log( "please input valid type and function" );
return;
}
var callbacks = this.cache[ type ] || [];
callbacks.push( func );
this.cache[ type ] = callbacks;
};
EventEmitter.off = function( type, func ){
if( !type ){
this.cache = {};
}else if( !func ){
delete this.cache[ type ];
}else{
var callbacks = this.cache[ type ] || [];
for( var i = 0; i < callbacks.length; ++i ){
callbacks[i] === func && callbacks.splice( i, 1 );
break;
}
if( !callbacks.length ){
delete this.cache[ type ];
}else{
this.cache[ type ] = callbacks;
}
}
};
EventEmitter.emit = function( type, context ){
if( !type ){
return;
}
context = context || {};
var callbacks = this.cache[ type ] || [];
var args = [].splice.call( arguments, 2, arguments.length - 2);
for( var i = 0; i < callbacks.length; ++i ){
callbacks[i].apply( context, args );
}
};
function serialize( data ){
var serializedFragments = [];
for( var key in data ){
var fragment = type.isString( data[key] ) ? data[key] : JSON.stringify( data[ key ] );
serializedFragments.push( key + "=" + fragment );
}
return serializedFragments.join( "&" );
}
function handleOptions( options ){
var defaultOptions = {
type : "GET" ,
async : true ,
context : this ,
jsonp : "callback" ,
crossDomain : false
};
options.type = options.type ? options.type.toUpperCase() : defaultOptions.type ;
options.async = options.async !== undefined ? !!options.async : defaultOptions.async ;
options.jsonp = options.jsonp ? options.jsonp : defaultOptions.jsonp;
options.context = options.context ? options.context : defaultOptions.context;
options.crossDomain = options.crossDomain !== undefined ? !!options.crossDomain : defaultOptions.crossDomain;
if( options.data ){
if( type.isObject( options.data ) ){
options.data = serialize( options.data );
}
}else{
options.data = null;
}
if( options.type === "GET" ){
if( options.url.indexOf( "?" ) == -1 ){
options.url += "?" + options.data;
}else{
options.url += "&" + options.data;
}
delete options.data;
}
return options;
}
function XHRFaker( transfer ){
this.mimeType = "";
this.isHandled = false;
this.jsonpName = "callback";
this.transfer = transfer();
this.request = transfer.request;
this.respond = transfer.respond;
this.preprocess = transfer.preprocess;
}
XHRFaker.prototype.setRequestHeader = function( header, name ){
this.transfer && this.transfer.setRequestHeader && this.transfer.setRequestHeader( header, name );
};
XHRFaker.prototype.getResponseHeader = function( header ){
if( this.transfer && this.transfer.getResponseHeader ){
return this.transfer.getResponseHeader( header ) || null;
}
return null;
};
XHRFaker.prototype.overridemimetype = function( mime ){
this.mimeType = mime;
this.transfer && this.transfer.overridemimetype && this.transfer.overridemimetype( mime );
};
XHRFaker.prototype.open = function( method, url, async, username, password ){
this.transfer && this.transfer.open && this.transfer.open( method, url, async, username, password );
};
XHRFaker.prototype.send = function( data ){
this.transfer && this.transfer.send && this.transfer.send( data );
};
XHRFaker.prototype.onreadystatechange = function( func, context ){
context = context || this;
this.transfer.onreadystatechange = function(){
func && func.call( context );
}
};
XHRFaker.prototype.getReadyState = function(){
if( this.transfer ){
return this.transfer.readyState || 0;
}
return 0;
};
XHRFaker.prototype.getStatus = function(){
if( this.transfer ){
var status = this.transfer.status;
if( status == 1223 || status == undefined ){
status = 204;
}
return status || null;
}
return null;
};
XHRFaker.prototype.getStatusText = function(){
if( this.transfer ){
return this.transfer.statusText || "";
}
return "";
};
XHRFaker.prototype.getResponseXML = function(){
if( this.transfer ){
return this.transfer.responseXML || "";
}
return "";
};
XHRFaker.prototype.getResponseText = function(){
if( this.transfer ){
return this.transfer.responseText || "";
}
return "";
};
XHRFaker.prototype.getResponse = function(){
if( this.transfer ){
return this.transfer.response || "";
}
return "";
};
var transferFactory = (function(){
var Transfers = {};
Transfers.xhr = function(){
var xhrObject;
var candidates = [
function(){ return new XMLHttpRequest(); },
function(){ return new ActiveXObject('MSXML2.XMLHTTP.6.0'); },
function(){ return new ActiveXObject('MSXML2.XMLHTTP.3.0'); },
function(){ return new ActiveXObject('Microsoft.XMLHTTP'); }
];
// IE7下的XMLHttpRequest访问本地文件的时候会报错
candidates[0] = !"1"[0] && window.location.protocol == "file:" ? function(){ throw new Error(""); } : candidates[0];
for( var i = 0; i < candidates.length; ++i ){
try{
xhrObject = candidates[i]();
xhr = candidates[i];
break;
}catch(e){}
}
return xhrObject;
};
Transfers.xhr.preprocess = function(){};
Transfers.xhr.request = function( options ){
if( options.username ){
this.open( options.type, options.url, options.async, options.username, options.password || "" );
}else{
this.open( options.type, options.url, options.async );
}
// 这个是用来告诉服务器,我们的请求是ajax的请求
this.setRequestHeader( "X-Requested-With", "XMLHttpRequest" );
if( options.headers ){
for( var header in options.headers ){
this.setRequestHeader( header, options.headers[ header ] );
}
}
this.overridemimetype( options.dataType || "text/plain" );
// 下面的代码是用来绑定事件的
this.onreadystatechange( this.respond );
this.send( options.type === "POST" && options.data ? options.data : null );
if( !options.async || this.getReadyState() == 4 ){
this.respond();
}
};
Transfers.xhr.respond = function(){
if( this.isHandled || this.getReadyState() != 4 ){
return;
}
var status = this.getStatus();
if( status >= 200 && status < 300 || status == 304 ){
EventEmitter.emit( "success" , this, this.getResponseText() );
EventEmitter.emit( "complete" , this, this.getResponseText() );
}else{
EventEmitter.emit( "error" , this, new Error());
EventEmitter.emit( "complete" , this, this.getResponseText() );
}
EventEmitter.off();
this.isHandled = true;
};
Transfers.script = function(){
return document.createElement( "script" );
};
Transfers.script.preprocess = function( options ){
};
Transfers.script.request = function( options ){
var self = this;
var script = this.transfer;
if( options.url.indexOf( "?" ) >= 0 ){
options.url += "&" + options.jsonp + "=" + options.jsonpCallback;
}else{
options.url += "?" + options.jsonp + "=" + options.jsonpCallback;
}
script.src = options.url;
if( script.readyState !== undefined && script.onerror !== null ){
script.onreadystatechange = function(){
if( this.readyState == "loaded" || this.readyState == "complete" ){
self.respond();
EventEmitter.emit( "complete", this, {} );
EventEmitter.off();
}
};
}else{
script.onerror = function(){
self.respond();
EventEmitter.emit( "error" , this, {} );
EventEmitter.emit( "complete" , this, {} );
EventEmitter.off();
};
script.onload = function(){
self.respond();
EventEmitter.emit( "success" , this, {} );
EventEmitter.emit( "complete" , this, {} );
EventEmitter.off();
};
}
document.body.appendChild( script );
};
Transfers.script.respond = function(){
if( this.isHandled ){
return;
}
this.transfer.onerror = this.transfer.onload = this.transfer.onreadystatechange = null;
document.body.removeChild( this.transfer );
this.isHandled = true;
};
return function( type ){
var transfer = null;
switch( type ){
case "xhr" :
transfer = Transfers.xhr;
break;
case "script" :
transfer = Transfers.script;
break;
}
return transfer;
};
})();
var http = function( options ){
if( !options.url ){
console.log( "url must to be specified" );
return;
}
options = handleOptions( options );
var events = [ "success", "complete", "error" ];
for( var i = 0; i < events.length; ++i ){
var event = events[i];
options[ event ] && type.isFunc( options[ event ] ) && EventEmitter.on( event, options[ event ] );
}
var transfer = null;
if( options.crossDomain ){
transfer = transferFactory( "script" ) ;
} else {
transfer = transferFactory( "xhr" );
}
var xhrFaker = new XHRFaker( transfer );
xhrFaker.preprocess( options );
xhrFaker.request( options );
};
return http;
}) | cc57a19fbb8f44efbe83b97fa94a7165b77063ea | [
"JavaScript"
] | 3 | JavaScript | wanglei20116527/front-end | 80f11cd728d0d0c546dbf0d14df2de11bfa8c0c0 | c0042b535b0d924136c98050a5cc059db624cf79 | |
refs/heads/master | <file_sep># Bootstrap - Pasja Informatyki
["Kurs Bootstrap #0 - Responsywność, frameworki front-endowe"](https://www.youtube.com/watch?v=KsUiRguudTs)<file_sep>package com.infoshare.academy.domain;
public class Castle {
private String name = "Asgard";
public Castle() {
}
}
<file_sep># HTML - Pasja Informatyki
["Kurs HTML. Tworzenie zawartości stron"](https://www.youtube.com/watch?v=1M0YXFW31hg&list=PLOYHgt8dIdox9Qq3X9iAdSVekS_5Vcp5r)<file_sep># Java podstawy - <NAME>
[Java Podstawy](https://www.youtube.com/channel/UCTLFvq9WIncYRxp5EfNPdLg)
<file_sep># Java Basics course
[Java Programming Basics](https://eu.udacity.com/course/java-programming-basics--ud282)<file_sep>package com.infoshare.academy;
import com.infoshare.academy.domain.Knight;
import com.infoshare.academy.domain.Quest;
import org.springframework.boot.CommandLineRunner;
import org.springframework.stereotype.Component;
@Component
public class Starter implements CommandLineRunner {
@Override
public void run(String... args) throws Exception {
Quest tutorialIsland = new Quest("Fisnish Tutorial Island");
Quest killTheDragon = new Quest("Kill the Green dragon");
Knight lancelot = new Knight("Lancelot", 23, tutorialIsland);
Knight percival = new Knight("Percival",31);
percival.setQuest(killTheDragon);
System.out.println(lancelot);
System.out.println(percival);
}
}
<file_sep>package junit.electricity;
import org.junit.Assert;
import org.junit.Test;
public class TwoElectricityMetersTest {
@Test
public void forNewMeterAddingFewKwhShouldReturnProperValue() {
//given
ElectricityMeter electricityMeter = new ElectricityMeter();
ElectricityMeter electricityMeter1 = new ElectricityMeter();
//when
electricityMeter.addKwh(1);
electricityMeter.addKwh(5);
electricityMeter.addKwh(2);
electricityMeter1.addKwh(4);
//then
Assert.assertTrue(electricityMeter.getKwh() == 8 && electricityMeter1.getKwh() == 4);
}
}<file_sep>class ToDo{
constructor(selector) {
this.toDoListContainer = document.querySelector(selector)
this.tasks = [{
taskName: '<NAME>',
isCompleted: true
}]
this.newTaskName = ''
this.render()
}
render(){
this.toDoListContainer.innerHTML = ''
this.buildUI()
this.renderTasksList()
}
renderTasksList(){
//you can use 'Array.prototype.forEach' instead of 'for loop'
for(let i=0; i<this.tasks.length; i++){
const taskContainer = document.createElement('div')
taskContainer.innerHTML = this.tasks[i].taskName
taskContainer.addEventListener(
'click',
() => this.onTaskClickHandler(i)
)
taskContainer.addEventListener(
'dblclick',
() => this.onTaskDoubleClickHandler(i)
)
if(this.tasks[i].isCompleted) taskContainer.style.textDecoration = 'line-through'
this.toDoListContainer.appendChild(taskContainer)
}
}
buildUI(){
const input = document.createElement('input')
const button = document.createElement('button')
button.innerHTML = 'Dodaj zadanie'
input.addEventListener(
'input',
(event) => this.onNewTaskNameChanged(event)
)
button.addEventListener(
'click',
() => this.onAddNewTaskClickHandler()
)
this.toDoListContainer.appendChild(input)
this.toDoListContainer.appendChild(button)
}
onTaskClickHandler(index){
this.tasks[index].isCompleted = !this.tasks[index].isCompleted
this.render()
}
//index tutaj to nr taska, więc usuwamy task z tym indeksem czyli zostawiamy inne, nierówne "i"
onTaskDoubleClickHandler(index){
this.tasks = this.tasks.filter((task, i) => index !== i)
this.render()
}
onNewTaskNameChanged(event){
this.newTaskName = event.target.value
}
onAddNewTaskClickHandler(){
this.addTask(this.newTaskName)
this.newTaskName = ''
this.render()
}
addTask(taskName) {
this.tasks = this.tasks.concat({
taskName: taskName,
isCompleted: false
})
this.render()
}
}
const toDo1 = new ToDo('div.toDo1')<file_sep># JavaScript ToDoList
Simple ToDoList actually without CSS style, made on iSA Workshop<file_sep># JavaCourse
My work of Java lessons along with front-end development for web applications
<file_sep>package lesson3kolekcje;
import java.util.HashMap;
import java.util.Map;
public class KolekcjeMap {
public static void main(String[] args) {
//Kolekcje - Mapa
//Bazuje na sposobie zapisu: klucz-wartość
System.out.println("*HashMap*");
Map<Integer, String> map = new HashMap<>();
//Klucze muszą być unikalne żeby nie stracić wartości
map.put(1,"jeden");
map.put(2,"dwa");
map.put(3,"trzy");
//Wyświetlanie HashMapy - iteracja poprzez gettery
for (Map.Entry<Integer, String> m : map.entrySet()){
int key = m.getKey();
String value = m.getValue();
System.out.println("Klucz: " + key + ", wartość: " + value);
}
System.out.println("Wyświetl wszystkie wartości: " + map.values());
System.out.println("Wyświetl wartość klucza nr 2: " + map.get(2));
}
}
<file_sep># Java Intermediate course - Strefa Kursów
[Kurs JAVA średniozaawansowany](https://strefakursow.pl/kursy/programowanie/kurs_java_sredniozaawansowany.html)<file_sep>package lesson6oop;
import java.util.Objects;
public class Tv {
private String make;
private Integer displaySize;
public Tv() {
}
public Tv(String make, Integer displaySize) {
this.make = make;
this.displaySize = displaySize;
}
public String getMake() {
return make;
}
public void setMake(String make) {
this.make = make;
}
public Integer getDisplaySize() {
return displaySize;
}
public void setDisplaySize(Integer displaySize) {
this.displaySize = displaySize;
}
public String description(){
StringBuilder sb = new StringBuilder();
sb.append("Tv make: ")
.append(make)
.append(", display size: ")
.append(displaySize)
.append("inch");
return sb.toString();
}
@Override
public String toString() {
return "Tv{" +
"make='" + make + '\'' +
", displaySize=" + displaySize +
'}';
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Tv tv = (Tv) o;
return Objects.equals(make, tv.make) &&
Objects.equals(displaySize, tv.displaySize);
}
@Override
public int hashCode() {
return Objects.hash(make, displaySize);
}
/* public void volumeUp(){
System.out.println("Volume up");
}
public void volumeDown(){
System.out.println("Volume down");
}
public void programUp(){
System.out.println("Program up");
}
public void programDown(){
System.out.println("Program down");
}*/
}
<file_sep><!DOCTYPE html>
<html lang="pl">
<head>
<meta charset="UTF-8">
<link rel="stylesheet" href="main.css">
<title>Strona główna</title>
</head>
<script language="JavaScript" type="text/javascript">
function zegar(){
d = new Date();
var h=d.getHours(),m=d.getMinutes(),s=d.getSeconds(),r;
r=(h<10?"0"+h:h)+":"+(m<10?"0"+m:m)+":"+(s<10?"0"+s:s);
document.getElementById('czas').innerHTML=r;
setTimeout("zegar()", 1000);
}
</script>
<body>
<div class="main-container">
<nav class="site-menu">
<ul class="site-nav">
<li class="site-nav-item">
<a href="index.html">Strona główna</a>
</li>
<li class="site-nav-item">
<a href="element1.html">Element1</a>
</li>
<li class="site-nav-item">
<a href="element2.html">Element2</a>
</li>
<li class="site-nav-item">
<a href="https://github.com/AreXe">Mój GitHub</a>
</li>
</ul>
</nav>
<div class="main-content">
<article>
<header>
<h1>Człowiek większość swojego życia spędza w mieszkaniu, dlatego też stanowi ono główny wyznacznik ludzkiego bytu. Mieszkanie służy zaspokojeniu podstawowych funkcji biologicznych i jest miejscem regeneracji.</h1>
<p>Napisał: AreXe</p>
</header>
<p>Rynek nieruchomości mieszkaniowych uzależniony jest od wpływu wielu czynników, zarówno ekonomicznych jak i społecznych. Zbadanie tych zależności może pomóc w planowaniu przyszłych inwestycji. W tym celu wykorzystano program STATISTICA, który za sprawą narzędzi analizy data mining pozwala utworzyć optymalny model prognostyczny.</p>
<figure>
<img src="images/MARS.PNG" alt="Prognozowanie liczby mieszkań metodą MARS">
<figcaption>Prognozowanie metodą MARS</figcaption>
<p>Tak dokładny wynik jest spowodowany predyktorami użytymi do budowy tego modelu, które stanowią sumę zmiennej zależnej v3. Obliczony model MARS1 nie może być uznany za prawidłowy, ponieważ nie spełnia on podstawowego warunku równania regresji. Należy dokonać obliczeń kolejnego modelu metodą MARSplines ze zredukowaną ilością zmiennych niezależnych. <q>Błąd MAPE dla tego modelu wynosi 2,38387%</q> - dodaje autor.
</p>
</figure>
</article>
<form>
<div>
<label for="nickname">Twój nick:</label>
</div>
<div>
<input type="text" id="nickname" name="nickname">
</div>
<div>
<label for="user-email">Twój e-mail:</label>
</div>
<div>
<input type="email" id="user-email" name="user-email" required>
</div>
<div>
<label for="content">Treść:</label>
</div>
<div>
<textarea rows="10" cols="50" id="content"
name="content" placeholder="Zapodaj komentarz :)"></textarea>
</div>
<div>
<input type="submit" value="dodaj">
</div>
</form>
</div>
<aside class="sidebar">
<div>Wstaw tu coś!</div>
</aside>
</div>
</body>
</html><file_sep>package lesson3kolekcje;
import java.util.ArrayList;
import java.util.List;
public class Kolekcje {
public static void main(String[] args) {
//*Kolekcja - ArrayList
System.out.println("*ArrayList*");
List<String> listaStr = new ArrayList<>();
//Dodawanie obiektów do ArrayList
listaStr.add("jeden");
listaStr.add("dwa");
listaStr.add("trzy");
/*
for (String str : listaStr) {
System.out.println(str);
}
*/
//Wyświetlenie obiektów w pętli
for(int i = 0; i < listaStr.size(); i++){
System.out.println(listaStr.get(i));
}
//Umieszczanie na liście kolejnych elementów w środku, nie tracimy elementu który tam był
listaStr.add(1,"zero");
for(int i = 0; i < listaStr.size(); i++){
System.out.println(listaStr.get(i));
}
System.out.println(listaStr.size());
//Usuwanie elementów z listy
listaStr.remove(1);
for(int i = 0; i < listaStr.size(); i++){
System.out.println(listaStr.get(i));
}
System.out.println(listaStr.size());
//Sprawdzenie czy obiekt znajduje się na liście
System.out.println(listaStr.contains("zero"));
//Sprawdzenie na jakim indexie znajduje się element
System.out.println(listaStr.indexOf("trzy"));
}
}
<file_sep># My first website
First steps in web design done with the book
["Moje pierwsza strona internetowa w HTML5 i CSS3"](http://ferrante.pl/books/html/book.pdf)<file_sep>package lesson5conditionals;
import java.util.Scanner;
public class Conditionals {
public static void main(String[] args) {
System.out.println("***If***");
StringBuilder name = new StringBuilder();
name.append("Ja");
name.append("nek");
String name2 = "Kasia";
if (name.equals("Jan") || name2.equals("Kasia")) {
System.out.println("Hi " + name + ", hi " + name2);
}
System.out.println("***Switch case***");
System.out.print("Choose the number: ");
Scanner scanner = new Scanner(System.in);
Integer x = scanner.nextInt();
switch (x) {
case 1:
showNumber(x);
break;
case 2:
showNumber(x);
break;
case 3:
showNumber(x);
break;
default:
System.out.println("Try with other number!");
break;
}
if (x == 4) {
System.out.println("X = 4");
}
}
public static void showNumber(int x) {
System.out.println("Your number is " + x);
}
}
<file_sep># Net Ninja HTML Tutorial
Easy steps into HTML
["HTML Tutorial for Beginners"](https://www.youtube.com/watch?v=Y1BlT4_c_SU&list=PL4cUxeGkcC9ibZ2TSBaGGNrgh4ZgYE6Cc&index=1)<file_sep><!DOCTYPE html>
<html lang="pl">
<head>
<meta charset="UTF-8">
<title>Oferta pracy: developer HTML i CSS</title>
</head>
<body>
<h1> Poszukujemy developera HTML i CSS</h1>
<img src="images/htmlcss.PNG" alt="Logo HTML i CSS">
<p>Poszukujemy <strong>zdolnego programisty</strong> posługującego się technologiami HTML i CSS. Oferujemy przyzwoitą formę zatrudnienia, wynagrodzenie dopasowane do potrzeb rynku i wiele benefitów!</p>
<p>Nie czekaj, napisz już dzisiaj! Ciepła posada jest w pełnej gotowości!</p>
<input type="text" value="wpisz tutaj tekst">
<a href="https://github.com/arexe" title="The story just starts">GitHub</a>
</body>
</html> | 953c7645757f896e5c99d2e9ddad131e7723f706 | [
"Markdown",
"Java",
"JavaScript",
"HTML"
] | 19 | Markdown | AreXe/JavaCourse | 86346799834a28f13bb7518d20af809e9850b49f | da510899291e06dfab11508da463fff130c14d2a | |
refs/heads/master | <repo_name>rach2812/python-third-assignment<file_sep>/README.md
# python-third-assignment
Final assignment for Introduction to Software Engineering
<file_sep>/a3/a3.py
"""
CSSE1001 Assignment 3
Semester 1, 2017
"""
import tkinter as tk
from tkinter import messagebox
import random
import model
import view
from game_regular import RegularGame
# # For alternative game modes
# from game_make13 import Make13Game
# from game_lucky7 import Lucky7Game
# from game_unlimited import UnlimitedGame
from highscores import HighScoreManager
__author__ = "<NAME>"
__email__ = "<EMAIL>"
__version__ = "1.0.2"
from base import BaseLoloApp
# Define your classes here
class LoloApp(BaseLoloApp):
"""Class for a Lolo game."""
def __init__(self, master, game=None, grid_view=None):
"""Constructor
Parameters:
master (tk.Tk|tk.Frame): The parent widget.
game (model.AbstractGame): The game to play. Defaults to a
game_regular.RegularGame.
grid_view (view.GridView): The view to use for the game. Optional.
Raises:
ValueError: If grid_view is supplied, but game is not.
"""
self._master = master
super().__init__(self._master, game=None, grid_view=None)
self._highscores = HighScoreManager()
self._master.title("LoLo :: {} Game".format(self._game.get_name()))
self._grid_view.pack_forget()
logo = LoloLogo(self._master)
logo.pack(side=tk.TOP, expand=True)
self._statusbar = StatusBar(self._master)
self._statusbar.pack(side=tk.TOP, expand=True, padx=10, pady=10)
self._statusbar.set_game(self._game.get_name())
self._grid_view.pack(side=tk.TOP, padx=10, pady=10)
self._lightning_count = 1
self._lightning_on = False
self._lightningBtn = tk.Button(self._master, text="Lightning ({})"
.format(self._lightning_count),
bg="white",command=self.toggle_lightning)
self._lightningBtn.pack(side=tk.TOP, expand=True, padx=10, pady=10)
menubar = tk.Menu(self._master)
self._master.config(menu=menubar)
filemenu = tk.Menu(menubar)
menubar.add_cascade(label="File", menu=filemenu)
filemenu.add_command(label="New Game", command=self.reset)
filemenu.add_command(label="Exit", command=self.exit)
self._master.bind_all("<Control-n>", self.shortcut_reset)
self._master.bind("<Control-l>", self.shortcut_toggle_lightning)
def set_name(self, name):
"""Gets the player's name from input entry on loading screen."""
self._player_name = name
def score(self, points):
"""Handles increase in score."""
self._statusbar.set_score(self._game.get_score())
self.add_lightning()
def toggle_lightning(self):
"""Toggles lightning ability (which removes a tile on activate) on and
off.
"""
if self._lightning_on:
self._lightning_on = False
self._lightningBtn.config(text="Lightning ({})".format
(self._lightning_count))
else:
self._lightning_on = True
self._lightningBtn.config(text="LIGHTNING ACTIVE ({})"
.format(self._lightning_count))
def shortcut_toggle_lightning(self, event):
"""Also toggles lightning ability, via a keyboard shortcut."""
self.toggle_lightning()
def check_lightning(self):
"""Checks if there are lightning available to use."""
if self._lightning_count == 0:
self._lightningBtn.config(text="Lightning ({})"
.format(self._lightning_count))
self._lightning_on = False
self._lightningBtn.config(state="disabled")
elif self._lightning_count > 0:
self._lightningBtn.config(state="normal")
if self._lightning_on:
self._lightningBtn.config(text="LIGHTNING ACTIVE ({})"
.format(self._lightning_count))
else:
self._lightningBtn.config(text="Lightning ({})"
.format(self._lightning_count))
def add_lightning(self):
"""Adds lightning to user's count."""
if self._game.get_score() % 10 == 0:
self._lightning_count += 1
self.check_lightning()
def reset(self):
"""Starts a new game of the same game type."""
self._lightning_count = 0
self._lightning_on = False
self._game.reset()
self._grid_view.draw(self._game.grid, self._game.find_connections())
def shortcut_reset(self, event):
"""Also starts a new game, using a keyboard shortcut."""
self.reset()
def activate(self, position):
"""Attempts to activate the tile at the given position.
Parameters:
position (tuple<int, int>): Row-column position of the tile.
Raises:
IndexError: If position cannot be activated.
"""
# Magic. Do not touch.
if position is None:
return
if self._game.is_resolving():
return
if position in self._game.grid:
if self._lightning_on:
self.remove(position)
self._lightning_count -= 1
self.check_lightning()
else:
if not self._game.can_activate(position):
messagebox.showerror(title="Oops!",
message=("Cannot activate position {}"
.format(position)))
def finish_move():
self._grid_view.draw(self._game.grid,
self._game.find_connections())
def draw_grid():
self._grid_view.draw(self._game.grid)
animation = self.create_animation(self._game.activate(position),
func=draw_grid,
callback=finish_move)
animation()
def game_over(self):
"""Handles game ending"""
if self._lightning_count == 0:
if self._game.game_over():
messagebox.showinfo(title="Game over!", message=
"There are no moves left. You scored: {}"
.format(self._game.get_score()))
self._highscores.record(self._game.get_score(),
self._game, self._set_name())
def exit(self):
"""Exits the app"""
self._master.destroy()
class StatusBar(tk.Frame):
"""A widget containg the score and game mode displays"""
def __init__(self, parent):
"""Widget that contails displays.
Constructor: StatusBar(tk.Widget)
Parameters:
parent (tk.Tk|tk.Toplevel): The parent widget.
"""
super().__init__(parent)
self._game_mode_name = tk.Label(self, text="Game", width=40)
self._game_mode_name.pack(side=tk.LEFT)
self._score = tk.Label(self, text="Score: ", width=40)
self._score.pack(side=tk.RIGHT)
def set_game(self, game_mode):
"""Change the game mode name label to show name of game."""
self._game_mode_name.config(text="{0} Game".format(game_mode))
def set_score(self, score):
"""Change the score label to show current score."""
self._score.config(text="Score: {}".format(score))
class LoloLogo(tk.Canvas):
"""A customised Canvas that displays the Lolo logo"""
def __init__(self, parent):
"""Create and initialise a screen.
Constructor: Screen(tk.Widget)
Parameters:
parent (tk.Frame|tk.Tk): The parent widget.
"""
super().__init__(parent, bg="white", width=400, height=150)
#Draw the first "L"
super().create_rectangle(50, 50, 80, 150, fill="purple", width=0)
super().create_rectangle(80, 120, 120, 150, fill="purple", width=0)
#Draw the first "o"
centre1 = (170, 115)
radius1 = 35
super().create_oval(centre1[0]-radius1, centre1[1]-radius1,
centre1[0]+radius1, centre1[1]+radius1,
fill="purple", width=0)
centre2 = (170, 115)
radius2 = 15
super().create_oval(centre2[0]-radius2, centre2[1]-radius2,
centre2[0]+radius2, centre2[1]+radius2,
fill="white", width=0)
#Draw the second "L"
super().create_rectangle(220, 50, 250, 150,
fill="purple", width=0)
super().create_rectangle(250, 120, 290, 150,
fill="purple", width=0)
#Draw the second "o"
centre3 = (340, 115)
radius3 = 35
super().create_oval(centre3[0]-radius3, centre3[1]-radius3,
centre3[0]+radius3, centre3[1]+radius3,
fill="purple", width=0)
centre4 = (340, 115)
radius4 = 15
super().create_oval(centre4[0]-radius4, centre4[1]-radius4,
centre4[0]+radius4, centre4[1]+radius4,
fill="white", width=0)
class AutoPlayingGame(BaseLoloApp):
"""A version of Lolo that automatically plays the game. Upon finish it
begins again with a new version."""
def __init__(self, master, game=None, grid_view=None):
"""Constructor
Parameters:
master (tk.Tk |tk.Frame): The parent widget.
game (mode.AbstractGame): The game to play. Defaults to a
game_regular.RegularGame.
grid_view(view.GridView): The view to use for the game. Optional.
Raises:
ValueError: If grid_view is supplied, but game is not.
"""
self._master = master
self._game = game
self._grid_view = grid_view
super().__init__(self._master, game=None, grid_view=None)
self._move_delay = 500
self._master.after(self._move_delay, self.move)
self._grid_view.pack_forget()
self._grid_view.pack(side=tk.TOP, padx=10, pady=10)
def bind_events(self):
"""Binds relevant events."""
self._game.on('resolve', self.resolve)
self._game.on('game_over', self._game.reset)
def resolve(self, delay=None):
"""Makes a move after a given delay."""
if delay is None:
delay = self._move_delay
self._master.after(delay, self.move)
def move(self):
"""Finds a connected tile randomly and activates it."""
connections = list(self._game.find_groups())
if connections:
# pick random valid move
cells = list()
for connection in connections:
for cell in connection:
cells.append(cell)
self.activate(random.choice(cells))
class LoadingScreen(tk.Frame):
"""Displays a loading screen with all the associated functionality."""
def __init__(self, master):
"""Constructor
Parameters:
master (tk.Tk|tk.Frame): The parent widget.
width: The width of the Frame.
height: The height of the Frame.
"""
super().__init__(master, bg="white", width=1000, height=800)
self._master = master
self._master.title("Lolo")
#Put in the logo
logo = LoloLogo(self._master)
logo.pack(side=tk.TOP, expand=True)
#Put in the name widget
name = tk.Frame(self._master)
name.pack(side=tk.TOP)
playernameLabel = tk.Label(name, text="Your name: ")
playernameLabel.pack(side=tk.LEFT)
self._playernameEntry = tk.Entry(name)
self._playernameEntry.pack(side=tk.LEFT)
self._playerName = self._playernameEntry.get()
#Create button
BUTTON_WIDTH = 40
buttons = tk.Frame(self._master)
buttons.pack(side=tk.LEFT, padx=20, pady=20)
playgameBtn = tk.Button(buttons, text="Play game", width=BUTTON_WIDTH,
command=self.start_game)
playgameBtn.pack(side=tk.TOP, expand=True, pady=50)
highscoresBtn = tk.Button(buttons, text="High scores",
width=BUTTON_WIDTH,
command=self.open_highscores)
highscoresBtn.pack(side=tk.TOP, expand=True, pady=50)
exitBtn = tk.Button(buttons, text="Exit game",
width=BUTTON_WIDTH, pady=50,
command=self.exit_game)
exitBtn.pack(side=tk.TOP, expand=True)
#Put in the auto playing game
auto_game_frame = tk.Frame(self._master)
auto_game_frame.pack(side=tk.RIGHT, padx=10, pady=10)
auto_game = AutoPlayingGame(auto_game_frame)
def start_game(self):
"""Begins a new game."""
root = tk.Toplevel(self)
app = LoloApp(root)
app.set_name(self._playerName)
root.mainloop()
def exit_game(self):
"""Exits the app."""
self._master.destroy()
def open_highscores(self):
"""Opens highscore window"""
highscore_window = tk.Toplevel(self)
highscore_window.title("Leaderboard")
highscores = Leaderboard(highscore_window)
class Leaderboard(tk.Frame):
"""Displays leaderboard including top ten high scorers, best player, and
static game representation of the best player's high scoring game.
Parameters:
master(tk.Tk|tk.Frame): The parent widget.
"""
def __init__(self, master):
super().__init__(master)
self._master = master
self._highscore = HighScoreManager()
highest = self._highscore.get_sorted_data()[0]
best_player = tk.Label(self._master, text=("Best Player: "+
highest['name'] + " with "+
str(highest['score'])))
best_player.pack(side=tk.TOP, expand=True)
grid_list = highest['grid']
self._game_grid = RegularGame.deserialize(grid_list)
HighScoreGame(self._master, game=RegularGame(types=3),
grid_view=self._game_grid)
#Create the leaderboard frame
players_list = tk.Frame(self._master, width=400)
players_list.pack(side=tk.TOP, padx=30, pady=30)
players_list_title = tk.Label(players_list, text="Leaderboard")
players_list_title.pack(side=tk.TOP, expand=True)
#Create the leaderboard entries
leader_name = tk.Frame(players_list)
leader_name.pack(side=tk.LEFT, expand=True, padx=40)
leader_score = tk.Frame(players_list)
leader_score.pack(side=tk.RIGHT, padx=40)
top_scorers = self._highscore.get_sorted_data()
for entry in top_scorers:
player_name = tk.Label(leader_name, text=(entry['name']))
player_name.pack(side=tk.TOP, anchor='w')
player_score = tk.Label(leader_score, text=(str(entry['score'])))
player_score.pack(side=tk.TOP, anchor='e')
class HighScoreGame(BaseLoloApp):
"""Static representation of the best player's highest scoring game."""
def __init__(self, master, game=None, grid_view=None):
"""Constructor
Parameters:
master (tk.Tk|tk.Frame): The parent widget.
game (model.AbstractGame): The game to play. Defaults to a
game_regular.RegularGame.
grid_view (view.GridView): The view to use for the game. Optional.
Raises:
ValueError: If grid_view is supplied, but game is not."""
self._master = master
self._game = game
self._grid_view = grid_view
super().__init__(self._master, game=None, grid_view=None)
def bind_events(self):
"""Binds relevant events."""
self._game.on('resolve', self.resolve)
self._game.on('game_over', self._game.reset)
def resolve(self, delay=None):
"""Makes a move after a given movement delay."""
if delay is None:
delay = self._move_delay
self._master.after(delay, self.move)
def move(self):
"""Finds a connected tile randomly and activates it."""
connections = list(self._game.find_groups())
if connections:
# pick random valid move
cells = list()
for connection in connections:
for cell in connection:
cells.append(cell)
self.activate(random.choice(cells))
def main():
"""Main function that runs the game."""
root = tk.Tk()
app = LoadingScreen(root)
root.mainloop()
if __name__ == "__main__":
main()
| d47b2ea15d890638430da930c4b55fe53d29cf40 | [
"Markdown",
"Python"
] | 2 | Markdown | rach2812/python-third-assignment | 7a52103372a9d566fac0ef072e2521a04327961f | 7b2d4ff2fcaa4a14bb6243f583deea8caa8cd452 | |
refs/heads/master | <repo_name>FaizanAkhtar0/Product-Recommender-API-FLASK<file_sep>/ProductRecommenderFlaskAPI/app.py
import pandas as pd
from flask import Flask, request, jsonify
from flask_restful import Api
from ProductRecommenderFlaskAPI.models.reviews import ReviewAndRatingPreferenceModel
from flask_cors import CORS, cross_origin
recommender = ReviewAndRatingPreferenceModel()
app = Flask(__name__)
app.config['CORS_HEADERS'] = 'Content-Type'
app.config['JSON_SORT_KEYS'] = False
cors = CORS(app, resources={r"/recommender": {"origins": "http://localhost:5000"}, r"/progress": {"origins": "http://localhost:5000"}})
# api = Api(app)
@app.route('/recommender', methods=['POST'])
@cross_origin(origin='localhost',headers=['Content- Type','Authorization'])
def get_data():
requested_data = request.get_json()
identifiers = list(requested_data['identifier'])
reviews = list(requested_data['review'])
ratings = list(requested_data['rating'])
ratings = [int(i) for i in ratings]
data = {'identifier': identifiers, 'review': reviews, 'rating': ratings}
df = pd.DataFrame(data)
statistics = recommender.recommendation(df=df)
return jsonify({'statistics': statistics}), 200
@app.route('/progress', methods=['GET'])
@cross_origin(origin='localhost',headers=['Content- Type','Authorization'])
def get_progress():
return jsonify({'progress': recommender.progress_percentage * 100})
if __name__ == "__main__":
app.run(port=5000, debug=True)
<file_sep>/ProductRecommenderFlaskAPI/models/reviews.py
from Analyzer.models_lib.sentiment_analyzer import SentimentAnalyzer
class ReviewAndRatingPreferenceModel:
_keys = [1, 2, 3, 4, 5]
_key_default_map = {
1: 0,
2: 0,
3: 0,
4: 0,
5: 0,
}
def __init__(self):
self._analyzer = SentimentAnalyzer()
self._df = None
self._review_label = []
self.progress_percentage = 0
def _fill_missing_keys(self, rating_counts_per_identifier):
present_keys = [k[0] for k in rating_counts_per_identifier]
for k in self._keys:
if k not in present_keys:
rating_counts_per_identifier.append((k, self._key_default_map.get(k)))
else:
return rating_counts_per_identifier
def map_rating_count_as_dict(self, rating_count_list):
converted_dict = {}
for item in rating_count_list:
converted_dict[str(item[0])] = item[1]
else:
return converted_dict.copy()
def extract_stats(self, df):
whole_progress_counter = len(df)
current_progress_counter = 0
stats = []
identifiers = df.identifier.unique()
for identifier in identifiers:
pos_count = 0
neg_count = 0
reviews_per_identifier = df.review[df.identifier == identifier]
ratings_per_identifier = df.rating[df.identifier == identifier]
for review in reviews_per_identifier:
prediction, confidence = self._analyzer.Sentiment(str(review), nlp=False)
if prediction == 'pos':
pos_count += 1
else:
neg_count += 1
current_progress_counter += 1
self.progress_percentage = round((current_progress_counter / whole_progress_counter), 2)
print("{:.3f}% - Complete".format(self.progress_percentage * 100))
else:
average_rating = (ratings_per_identifier.sum() / len(ratings_per_identifier))
rating_counts_per_identifier = [(
ratings_per_identifier.value_counts().index[i], list(ratings_per_identifier.value_counts())[i]
) for i in range(len(ratings_per_identifier.value_counts()))]
rating_counts_per_identifier = self._fill_missing_keys(rating_counts_per_identifier)
rating_counts_per_identifier.sort(key=lambda x: x[0], reverse=True)
rating_counts_per_identifier = self.map_rating_count_as_dict(rating_counts_per_identifier)
total_rating_count = len(ratings_per_identifier)
stats.append((
identifier, len(reviews_per_identifier), pos_count, neg_count, total_rating_count, round(average_rating, 1),
rating_counts_per_identifier
))
else:
return stats
def recommendation(self, df):
statistics = {}
stats = self.extract_stats(df=df)
stats.sort(key=lambda x: x[5], reverse=True)
for stat in stats:
identifier, total_reviews, pos_review_count, neg_review_count, total_rating_count, \
avg_rating, rating_counts = stat
history = {
'total_reviews': total_reviews, 'positive_review_count': pos_review_count,
'negative_review_count': neg_review_count, 'total_rating_count': total_rating_count,
'average_rating': avg_rating, 'category_rating_counts': rating_counts
}
statistics[identifier] = history.copy()
else:
return statistics.copy()
| 115fbe4b11b34d7588b809aaa66cade7afc9cec9 | [
"Python"
] | 2 | Python | FaizanAkhtar0/Product-Recommender-API-FLASK | 975e9ad8e324ea61ebba0aa6a5265caa734a72d6 | 00bc727052ed69c567ce32601c1d2fdbbf233f5f | |
refs/heads/master | <repo_name>eltoncezar/Album-Art-Folder-Icon<file_sep>/ecr.AlbumFolderIcon/Utils/ImageUtils.cs
//http://jasonjano.wordpress.com/2010/02/13/image-resizing-and-cropping-in-c/
//http://tech.pro/tutorial/620/csharp-tutorial-image-editing-saving-cropping-and-resizing
using System;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Drawing.Imaging;
using System.IO;
using System.Linq;
using System.Windows.Media.Imaging;
namespace ecr.AlbumFolderIcon.Utils
{
public class ImageUtils
{
#region Cropping
//Overload for crop that default starts top left of the image.
public static System.Drawing.Image CropImage(System.Drawing.Image Image, int Height, int Width)
{
return CropImage(Image, Height, Width, 0, 0);
}
//The crop image sub
public static System.Drawing.Image CropImage(System.Drawing.Image Image, int Height, int Width, int StartAtX, int StartAtY)
{
Image outimage;
MemoryStream mm = null;
try
{
//check the image height against our desired image height
if (Image.Height < Height)
{
Height = Image.Height;
}
if (Image.Width < Width)
{
Width = Image.Width;
}
//create a bitmap window for cropping
Bitmap bmPhoto = new Bitmap(Width, Height, PixelFormat.Format24bppRgb);
bmPhoto.SetResolution(72, 72);
//create a new graphics object from our image and set properties
Graphics grPhoto = Graphics.FromImage(bmPhoto);
grPhoto.SmoothingMode = SmoothingMode.AntiAlias;
grPhoto.InterpolationMode = InterpolationMode.HighQualityBicubic;
grPhoto.PixelOffsetMode = PixelOffsetMode.HighQuality;
//now do the crop
grPhoto.DrawImage(Image, new Rectangle(0, 0, Width, Height), StartAtX, StartAtY, Width, Height, GraphicsUnit.Pixel);
// Save out to memory and get an image from it to send back out the method.
mm = new MemoryStream();
bmPhoto.Save(mm, System.Drawing.Imaging.ImageFormat.Jpeg);
Image.Dispose();
bmPhoto.Dispose();
grPhoto.Dispose();
outimage = Image.FromStream(mm);
return outimage;
}
catch (Exception ex)
{
throw new Exception("Error cropping image, the error was: " + ex.Message);
}
}
#endregion Cropping
#region Resizing
//Hard resize attempts to resize as close as it can to the desired size and then crops the excess
public static System.Drawing.Image HardResizeImage(int Width, int Height, System.Drawing.Image Image)
{
int width = Image.Width;
int height = Image.Height;
Image resized = null;
if (Width > Height)
{
resized = ResizeImage(Width, Width, Image);
}
else
{
resized = ResizeImage(Height, Height, Image);
}
Image output = CropImage(resized, Height, Width);
//return the original resized image
return output;
}
//Image resizing
public static System.Drawing.Image ResizeImage(int maxWidth, int maxHeight, System.Drawing.Image Image)
{
int width = Image.Width;
int height = Image.Height;
//if (width > maxWidth || height > maxHeight)
//{
//The flips are in here to prevent any embedded image thumbnails -- usually from cameras
//from displaying as the thumbnail image later, in other words, we want a clean
//resize, not a grainy one.
Image.RotateFlip(System.Drawing.RotateFlipType.Rotate180FlipX);
Image.RotateFlip(System.Drawing.RotateFlipType.Rotate180FlipX);
float ratio = 0;
if (width > height)
{
ratio = (float)width / (float)height;
width = maxWidth;
height = Convert.ToInt32(Math.Round((float)width / ratio));
}
else
{
ratio = (float)height / (float)width;
height = maxHeight;
width = Convert.ToInt32(Math.Round((float)height / ratio));
}
//return the resized image
return Image.GetThumbnailImage(width, height, null, IntPtr.Zero);
//}
//return the original resized image
//return Image;
}
#endregion Resizing
#region Converters
public static BitmapImage ConvertImageToBitmapImage(Image img)
{
// Convert the image in resources to a Stream
Stream ms = new MemoryStream();
img.Save(ms, ImageFormat.Png);
// Create a BitmapImage with the Stream.
BitmapImage bitmap = new BitmapImage();
bitmap.BeginInit();
bitmap.StreamSource = ms;
bitmap.EndInit();
return bitmap;
}
public static BitmapImage ConvertIconToBitmapImage(Icon icon)
{
// Convert the image in resources to a Stream
Stream ms = new MemoryStream();
icon.Save(ms);
// Create a BitmapImage with the Stream.
BitmapImage bitmap = new BitmapImage();
bitmap.BeginInit();
bitmap.StreamSource = ms;
bitmap.EndInit();
return bitmap;
}
public static BitmapImage ConvertBitmapToBitmapImage(Bitmap bitmap)
{
using (MemoryStream memory = new MemoryStream())
{
bitmap.Save(memory, ImageFormat.Png);
memory.Position = 0;
BitmapImage bitmapImage = new BitmapImage();
bitmapImage.BeginInit();
bitmapImage.StreamSource = memory;
bitmapImage.CacheOption = BitmapCacheOption.OnLoad;
bitmapImage.EndInit();
return bitmapImage;
}
}
#endregion Converters
}
}<file_sep>/README.md
# Album Art Folder Icon
Album Art Folder Icon is a helper to organize your collections of movies/music.
It turns your Windows 7/8 boring folder icons into amazing XP Style folder icons!
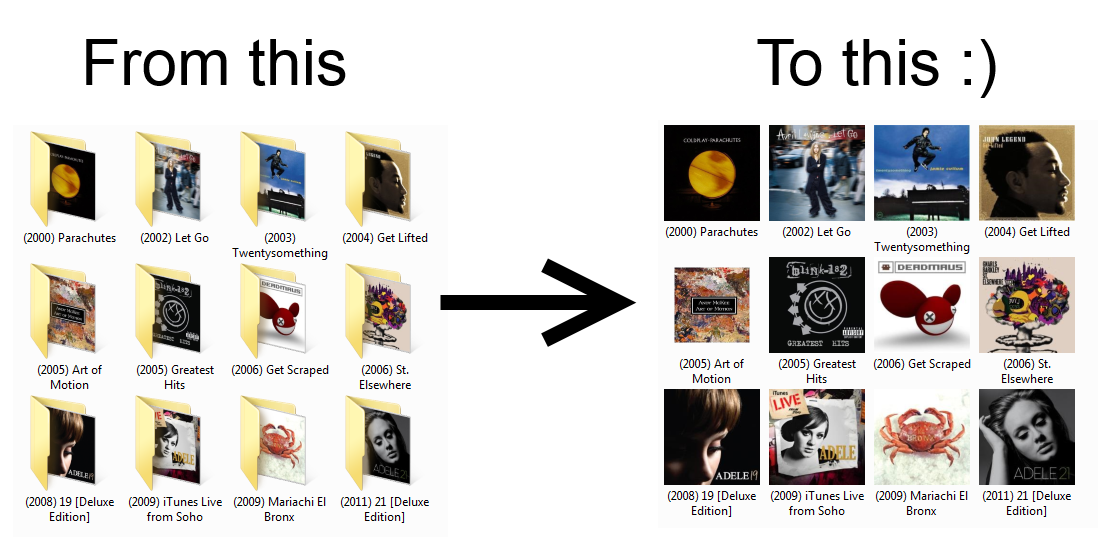
# [Download](https://github.com/eltoncezar/Album-Art-Folder-Icon/releases/download/v1.0/Album.Art.Folder.Icon.exe)
<file_sep>/ecr.AlbumFolderIcon/MainWindow.xaml.cs
using System;
using System.Collections.Specialized;
using System.Drawing;
using System.IO;
using System.Linq;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Forms;
using ecr.AlbumFolderIcon.Core;
using ecr.AlbumFolderIcon.Utils;
namespace ecr.AlbumFolderIcon
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
private StringCollection log = new StringCollection();
public MainWindow()
{
InitializeComponent();
rtbxErrors.HorizontalScrollBarVisibility = ScrollBarVisibility.Auto;
rtbxErrors.Document.PageWidth = 1000;
}
private void btnSearchPath_Click(object sender, RoutedEventArgs e)
{
FolderBrowserDialog dialog = new FolderBrowserDialog();
DialogResult result = dialog.ShowDialog();
if (result == System.Windows.Forms.DialogResult.OK)
txtPath.Text = dialog.SelectedPath;
}
private void btnStart_Click(object sender, RoutedEventArgs e)
{
//TODO: Insert validation here
if (!Directory.Exists(txtPath.Text))
{
System.Windows.Forms.MessageBox.Show("Directory doesn't exist.");
return;
}
Main(txtPath.Text);
}
public void Main(string path)
{
DirectoryInfo rootDir = new DirectoryInfo(path);
WalkDirectoryTree(rootDir);
// Write out all the files that could not be processed.
rtbxErrors.AppendText("\r\r Files with error: \r");
foreach (string s in log)
{
rtbxErrors.AppendText(s + "\r");
}
rtbxErrors.ScrollToEnd();
}
public void WalkDirectoryTree(DirectoryInfo root)
{
FileInfo[] files = null;
DirectoryInfo[] subDirs = null;
string folderPath = root.FullName + "\\" + txbFileName.Text + txbFileExtension.Text;
if (File.Exists(folderPath))
{
// Update the folder attributes
DirectoryInfo dir = new DirectoryInfo(root.FullName);
dir.Attributes = dir.Attributes | FileAttributes.System;
CreateIcon(folderPath);
CreateDesktopIni(root.FullName);
rtbxErrors.AppendText(root.FullName + "\r");
}
if (ckbIncludeSubfolders.IsChecked == true)
{
// Now find all the subdirectories under this directory.
subDirs = root.GetDirectories();
foreach (System.IO.DirectoryInfo dirInfo in subDirs)
{
// Resursive call for each subdirectory.
WalkDirectoryTree(dirInfo);
}
}
}
public void CreateIcon(string path)
{
FileInfo file = new FileInfo(path);
string fileName = file.DirectoryName + "\\folder.ico";
if (!File.Exists(fileName))
{
try
{
System.Drawing.Image src = System.Drawing.Image.FromFile(path);
ImageOrientation orientation = src.Height < src.Width ? ImageOrientation.Landscape : ImageOrientation.Portrait;
int size = orientation == ImageOrientation.Landscape ? src.Height : src.Width;
int sizeToCrop = orientation == ImageOrientation.Landscape ? src.Width - src.Height : src.Height - src.Width;
System.Drawing.Image croppedImage = ImageUtils.CropImage(src, size, size, (orientation == ImageOrientation.Landscape ? sizeToCrop / 2 : 0), (orientation == ImageOrientation.Portrait ? sizeToCrop / 2 : 0));
System.Drawing.Image resizedImage = ImageUtils.ResizeImage(256, 256, croppedImage);
Icon icon = System.Drawing.Icon.FromHandle(new Bitmap(resizedImage).GetHicon());
IconEx Iconex = new IconEx(icon);
Iconex.Items.RemoveAt(0);
// 256x256
IconDeviceImage iconDeviceImage = new IconDeviceImage(new System.Drawing.Size(256, 256), System.Windows.Forms.ColorDepth.Depth32Bit);
iconDeviceImage.IconImage = new Bitmap(ImageUtils.ResizeImage(256, 256, resizedImage));
Iconex.Items.Add(iconDeviceImage);
// 48x48
iconDeviceImage = new IconDeviceImage(new System.Drawing.Size(48, 48), System.Windows.Forms.ColorDepth.Depth32Bit);
iconDeviceImage.IconImage = new Bitmap(ImageUtils.ResizeImage(48, 48, resizedImage));
Iconex.Items.Add(iconDeviceImage);
// 32x32
iconDeviceImage = new IconDeviceImage(new System.Drawing.Size(32, 32), System.Windows.Forms.ColorDepth.Depth32Bit);
iconDeviceImage.IconImage = new Bitmap(ImageUtils.ResizeImage(32, 32, resizedImage));
Iconex.Items.Add(iconDeviceImage);
// 24x24
iconDeviceImage = new IconDeviceImage(new System.Drawing.Size(24, 24), System.Windows.Forms.ColorDepth.Depth32Bit);
iconDeviceImage.IconImage = new Bitmap(ImageUtils.ResizeImage(24, 24, resizedImage));
Iconex.Items.Add(iconDeviceImage);
// 16x16
iconDeviceImage = new IconDeviceImage(new System.Drawing.Size(16, 16), System.Windows.Forms.ColorDepth.Depth32Bit);
iconDeviceImage.IconImage = new Bitmap(ImageUtils.ResizeImage(16, 16, resizedImage));
Iconex.Items.Add(iconDeviceImage);
Iconex.Save(fileName);
// "Hide" the folder.ico
File.SetAttributes(fileName, File.GetAttributes(fileName) | FileAttributes.Hidden);
//imgRect.Source = ImageUtils.ConvertImageToBitmapImage(resizedImage);
}
catch (Exception)
{
log.Add(path);
}
}
}
public void CreateDesktopIni(string path)
{
string fileName = path + "\\Desktop.ini";
if (!File.Exists(fileName))
{
try
{
StringBuilder str = new StringBuilder();
str.AppendLine("[.ShellClassInfo]");
str.AppendLine("IconResource=folder.ico,0");
File.WriteAllText(fileName, str.ToString());
// "Hide" the desktop.ini
File.SetAttributes(fileName, File.GetAttributes(fileName) | FileAttributes.Hidden);
}
catch (Exception)
{
log.Add(path);
}
}
}
}
public enum ImageOrientation
{
Landscape,
Portrait
}
} | a688f79e6abbb4eec252d44050db9f439bbda351 | [
"Markdown",
"C#"
] | 3 | C# | eltoncezar/Album-Art-Folder-Icon | 94554d321b057d168b47859ac65333ef83d331e8 | fd21c55700384e64958fc66fac3a2af7b92df8eb | |
refs/heads/master | <file_sep>from BaseHTTPServer import BaseHTTPRequestHandler, HTTPServer
from os import curdir, sep
import cgi
PORT_NUMBER = 8080
#class for request handling
class myHandler(BaseHTTPRequestHandler):
#handler for GET request
def do_GET(self):
if self.path=="/":
self.path="/response.html"
try:
f = open(curdir + sep + self.path)
self.send_response(200) #add status code
self.send_header('Content-type', 'text/html') #proper mimetype
self.end_headers() #close headers
self.wfile.write(f.read()) #add file to response
f.close()
except IOError:
self.send_error(404,'File Not Found: %s' % self.path)
#starting server script
try:
#create server instance
server = HTTPServer(('', PORT_NUMBER), myHandler)
print 'Started server on port ' , PORT_NUMBER
#run server
server.serve_forever()
except KeyboardInterrupt:
print 'KeyboardInterrupt received, shutting server down'
server.socket.close()<file_sep>
<head>
<title>Head</title>
</head>
<body>
<h1> Body: </h1>
<ol>
<li>Wed Dec 21 08:55:28 2016</li>
<li>Wed Dec 21 08:55:29 2016</li>
<li>Wed Dec 21 08:55:34 2016</li>
<li>Wed Dec 21 08:55:35 2016</li>
<li>Wed Dec 21 08:55:37 2016</li>
<li>Wed Dec 21 08:55:38 2016</li>
<li>Wed Dec 21 08:55:39 2016</li>
</ol>
</body>
<file_sep>import os
FILE = 'response.html'
class Response:
def __init__(self):
self.file = os.open(os.curdir + os.sep + FILE, os.O_RDWR|os.O_CREAT)
os.ftruncate(self.file, 0)
os.lseek(self.file, 0, os.SEEK_SET)
os.write(self.file,
"""
<head>
<title>Head</title>
</head>
<body>
<h1> Body: </h1>
<ol>
</ol>
</body>
"""
)
def up(self,time):
os.lseek(self.file, -14, os.SEEK_END)
os.write(self.file, " <li>" + time +
"""</li>
</ol>
</body>
"""
)
| 8be3e72c6799514f639821a516db4fa7930abed4 | [
"Python",
"HTML"
] | 3 | Python | RobertSzz/simple-python-server | f7eaed7b9c6b4d8a09f8f5da56d834de5eed1f74 | 7a6897d8afff520c7398550b5465cdc9c9953413 | |
refs/heads/master | <repo_name>yanagao/react-demo<file_sep>/build/testUtilities.js
var ReactTestUtils = require('react-addons-test-utils');<file_sep>/README.md
# react-demo
=================================================
### 链接
1、https://yanagao.github.io/react-demo/index.html <br/>
2、https://yanagao.github.io/react-demo/index2.html <br/>
3、https://yanagao.github.io/react-demo/lifeCircle.html <br/>
4、https://yanagao.github.io/react-demo/loading.html <br/>
5、https://yanagao.github.io/react-demo/twoWayBinding.html <br/>
6、https://yanagao.github.io/react-demo/animation.html <br/> | fe22c8f996bd6fc618af1901c95bc78f16172943 | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | yanagao/react-demo | 8eb44d3d95123e6b816c742a665091f39339e4eb | e37fe5e3e749ebdf87bbeb3628c22e73cd2b74f8 | |
refs/heads/master | <file_sep>---
layout: post
title: "Site is Launched!"
date: 2016-08-21
---
After multiple attempts of creating a personal website, I have finally followed through. My previous attempts were way too complicated and made it hard to be productive and proactive. I tried making a single-page application (SPA) with React and Redux, but it was too hard to make changes and I didn't make smart decisions with deployment. I also tried making a full stack Flask/Jinja website, but again, deployment troubles kept me from making progress.
Now, it is _very_ easy to work with my website. It's hosted completely by [GitHub Pages](https://pages.github.com) and powered by [Jekyll](http://jekyllrb.com), so I don't have to worry about deployments or overly complicated JavaScript frameworks. All I have to do is write, because it's Markdown! Jekyll is super easy to use and manage, so I can make rapid changes with little-to-no friction. I look forward to writing more and publishing content. Check back frequently!
--Nick
<file_sep>---
layout: default
title: <NAME>
---
<section class="section">
<div class="container has-text-centered">
<figure class="image nick-pic">
<img src="/assets/images/nick.jpg">
</figure>
<h1 class="title">Hi there, I'm <NAME>!</h1>
<h2 class="subtitle">I'm a software developer. <a href="/about">Read more about me...</a></h2>
</div>
</section>
<file_sep># ncbrown1.github.io<file_sep>#!/bin/bash
echo "Building site..."
bundle exec jekyll build
git add docs
git commit -m"Automatic site build :: $(date)"
git push origin master
echo "Deployed site to GitHub Pages."
<file_sep><!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>About Nick</title>
<meta name="description" content="">
<meta name="author" content="<NAME> (<EMAIL>)">
<!-- Mobile Specific Metas
–––––––––––––––––––––––––––––––––––––––––––––––––– -->
<meta name="viewport" content="width=device-width, initial-scale=1">
<!-- FONT
–––––––––––––––––––––––––––––––––––––––––––––––––– -->
<link href="//fonts.googleapis.com/css?family=Raleway:400,300,600" rel="stylesheet" type="text/css">
<!-- CSS
–––––––––––––––––––––––––––––––––––––––––––––––––– -->
<link rel="stylesheet" type="text/css" href="/assets/css/main.css">
<!-- JS
–––––––––––––––––––––––––––––––––––––––––––––––––– -->
<script src="/assets/js/font-awesome.js"></script>
<!-- Favicon
–––––––––––––––––––––––––––––––––––––––––––––––––– -->
<link rel="icon" type="image/png" href="/assets/images/favicon.png">
</head>
<body>
<!--BEGIN Nav Header -->
<section class="hero is-primary is-bold">
<!-- Hero header: will stick at the top -->
<div class="hero-head">
<header class="nav">
<div class="container">
<div class="nav-left">
<a class="nav-item is-brand" href="/">
<h1 class="title is-4">Nick Brown</h1>
</a>
</div>
<span class="nav-toggle">
<span></span>
<span></span>
<span></span>
</span>
<div class="nav-right nav-menu">
<a class="nav-item" href="/">Home</a>
<a class="nav-item" href="/about">About</a>
<a class="nav-item" href="/resume">Resume</a>
<a class="nav-item" href="/resume/portfolio">Portfolio</a>
<a class="nav-item" href="/blog">Blog</a>
</div>
</div>
</header>
</div>
<!-- Hero content: will be in the middle -->
<div class="hero-body">
<div class="container has-text-centered">
<h1 class="title">
About Nick
</h1>
<h2 class="subtitle">
How I got here and why I made this blog.
</h2>
</div>
</div>
<!-- Hero footer: will stick at the bottom -->
<div class="hero-foot">
<nav class="tabs is-boxed is-fullwidth sideways-scroll">
<div class="container">
<ul>
<li><a href="#my-blog">My Blog</a></li>
<li><a href="#my-self">My Self</a></li>
<li><a href="#my-story">My Story</a></li>
<li><a href="/about/books">My Books</a></li>
</ul>
</div>
</nav>
</div>
</section>
<!--END Nav Header -->
<section class="section">
<div class="container content">
<h2 class="title is-3" id="my-blog">My Blog</h2>
<p>This blog is the manifestation of the ongoings in my head that normally only get shared verbally with those that I interact with. I often find myself repeating the same stories, lessons, and ideas to many different people in a week, so perhaps it is time to start keeping track of it all in a way that is public for more than just my friends.</p>
<p>What you read here will be a mix of material based on what is interesting to me at any given time. Most of the time, it will be programming or software-related, but it may venture out to different subjects, such as business, lifestyle, or even music. I have not blogged before, so please be patient as I develop my online voice.</p>
<h2 class="title is-3" id="my-self">My Self</h2>
<p>My first line of code was written a little over six years ago in my AP Computer Science class in high school: “hello world” in Java. It was a simple idea planted in my head, that I could just type some words in a text file and make the computer do what I wanted it to do. That idea took off and I just hung on for the ride. Hundreds of “hello world” programs later, I sit here about to start my career in the tech industry as a software developer.</p>
<p>I am close to graduating from UC Santa Barbara with my Master’s degree in Computer Science, and my career is starting to unfold itself before my eyes. I do not know what the next few years have in store for me, but all I hope for is that I will be able to continue to learn and grow professionally so that I can continue to provide value to those around me. My latest endeavor has been Site Reliability Engineering at LinkedIn, where I am learning a great deal about product operations and site stability for massive scale cloud applications. It is an extremely rewarding experience, and it is my plan to continue with it.</p>
<h2 class="title is-3" id="my-story">My Story</h2>
<p>I was born and raised in Orange County, California, where I completed all of my K-12 schooling. My alma mater there is Valencia High School (class of 2013), where I participated in music, academic decathlon, student leadership, and ValTech (their tech program). Once I graduated from high school, I transitioned to college at UC Santa Barbara in Goleta, CA, where I studied Computer Science. I completed my Bachelor’s of Science in two and a half years, but was allowed to continue on into a Master’s program right away.</p>
<p>When I am not coding, I really enjoy listening to and playing music. I am an avid saxophonist in the UCSB Jazz ensembles, which helps me exercise my creativity in a less engineer-minded way. Hopefully I will be able to continue with that past graduation. To a lesser extent, I enjoy playing guitar and ukelele, but it seems as though my skills at those instruments have deteriorated over time.</p>
<p>Aside from music, my other passions include cooking/food, reading**, entrepreneurship, and fitness.</p>
<p>** If you want to know what I read, check out my "<a href="/about/books">Books to Read</a>" page!</p>
</div>
</section>
<!--BEGIN Footer -->
<footer class="footer">
<div class="container">
<div class="content has-text-centered">
<p>
<strong>Contact</strong> or <strong>Follow</strong> me on these platforms:
</p>
<p>
<a href="mailto:<EMAIL>" title="email">
<i class="fa fa-envelope-o fa-2x" aria-hidden="true"></i>
</a> _
<a href="https://github.com/ncbrown1" title="github">
<i class="fa fa-github-alt fa-2x" aria-hidden="true"></i>
</a> _
<a href="https://linkedin.com/in/ncbrown1" title="linkedin">
<i class="fa fa-linkedin fa-2x" aria-hidden="true"></i>
</a> _
<a href="https://twitter.com/ncbrown1" title="twitter">
<i class="fa fa-twitter fa-2x" aria-hidden="true"></i>
</a> _
<a href="https://open.spotify.com/user/121577201" title="spotify">
<i class="fa fa-spotify fa-2x" aria-hidden="true"></i>
</a>
</p>
<p>
<a href="http://nick-brown.me"><NAME></a> © 2016
</p>
</div>
</div>
</footer>
<!--END Footer -->
<script type="text/javascript" src="/assets/js/jquery.min.js"></script>
<script type="text/javascript">
$(function() {
$(".nav-toggle").on("click", function(event) {
$(".nav-menu").toggleClass('is-active');
});
});
</script>
<script>
(function(i,s,o,g,r,a,m){i['GoogleAnalyticsObject']=r;i[r]=i[r]||function(){
(i[r].q=i[r].q||[]).push(arguments)},i[r].l=1*new Date();a=s.createElement(o),
m=s.getElementsByTagName(o)[0];a.async=1;a.src=g;m.parentNode.insertBefore(a,m)
})(window,document,'script','https://www.google-analytics.com/analytics.js','ga');
ga('create', 'UA-69460199-2', 'auto');
ga('send', 'pageview');
</script>
</body>
</html>
| 050b7374dc93c89252b99081a1a2d15b384e33d5 | [
"Markdown",
"HTML",
"Shell"
] | 5 | Markdown | ncbrown1/ncbrown1.github.io | 02eff114c65c9ddf7b3a14580627313f496b1283 | 89df92b3db67f986d6f253c63f3872aa034c36bb | |
refs/heads/master | <repo_name>Tsui89/qcloud<file_sep>/health_mysql.sh
netstat -anp |grep 8306
<file_sep>/readme.md
```code
elasticsearch:
cluster-nodes: {{range $dir := lsdir "/hosts/postgres"}}{{$ip := printf "/hosts/postgres/%s/ip" $dir}}{{getv $ip}}{{end}}:8200
work-order:
index: k2fuxi_wo
type: wo
es-import-ticket: c1c3fad1-1b09-4693-82fe-904541d794a5
minimum-should-match: 90%
spring:
datasource:
name: search-ds
url: jdbc:mysql://{{range $dir := lsdir "/hosts/mysql"}}{{$ip := printf "/hosts/mysql/%s/ip" $dir}}{{getv $ip}}{{end}}:8306/db_k2fuxi_wo?useSSL=false&useUnicode=true&characterEncoding=utf8
username: root
password: <PASSWORD>
driver-class-name: com.mysql.jdbc.Driver
wo-import-script:
shell-path: /home/ubuntu/python/woImport.sh
eureka:
client:
serviceUrl:
defaultZone: http://127.0.0.1:8000/eureka/
```
```
window['__API__'] = 'http://{{getv "/host/ip"}}:9000/k2fuxi-search/'
window['__LOGINAPI__'] = 'http://{{getv "/host/ip"}}:9000/k2fuxi-uc/'
window['__CORPUSAPI__'] = 'http://{{getv "/host/ip"}}:9000/k2fuxi-corpus/'
window['__STATISTICSAPI__'] = 'http://{{getv "/host/ip"}}:9000/k2fuxi-statistics/'
```
```
,{
"key": "service_params",
"description": "Custom service configuration properties",
"type": "array",
"properties": [{***
"key": "role_name",
"description": "Custom service the role (role_name) configuration properties",
"type": "array",
"properties": [{***
"key": "param",
"label": "Role Param",
"description": "The param for all slave nodes",
"type": "string",
"pattern": "^value.+",
"default": "value1",
"range": ["value1", "value11"],
"required": "yes"
}]
}]
}
```
,
{
"key": "env",
"description": "Custom service configuration properties",
"type": "array",
"properties": [
{
"key": "role_name",
"description": "Custom service the role (role_name) configuration properties",
"type": "array",
"properties": [
{
"key": "param",
"label": "Role Param",
"description": "The param for all slave nodes",
"type": "integer",
"changeable": true,
"default": "8080",
"min": 8000,
"max": 30000,
"required": "yes"
}
]
}
]
}<file_sep>/health_check.py
#!/usr/bin/env python
import urllib
import sys
try:
data = urllib.urlopen('http://localhost:9002/health').read()
except:
sys.exit(-1)
else:
if "UNKNOWN" in data:
sys.exit(-1)
else:
sys.exit(0)<file_sep>/instruction.md
1.服务条款的确认和接纳
本网站及APP的各项内容和服务的所有权归本公司拥有。用户在接受本服务之前,请务必仔细阅读本条款。用户使用服务,或通过完成注册程序,表示用户接受所有服务条款。
2.用户同意:
- 提供及时、详尽及准确的个人资料。
- 不断更新注册资料、符合及时、详尽、准确的要求。
如果用户提供的资料不准确,本网站有结束服务的权利。
本网站及APP将不公开用户的姓名、地址、电子邮箱、帐号和电话号码等信息(请阅隐私保护条款)。
用户在本网站和APP的任何行为必须遵循:
(1) 传输资料时必须符合中国有关法规。
(2) 使用信息服务不作非法用途和不道德行为。
(3) 不干扰或混乱网络服务。
(4) 遵守所有使用服务的网络协议、规定、程序和惯例。用户的行为准则是以因特网法规,政策、程序和惯例为根据的。
3.服务条款的修改
本网站及APP有权在必要时修改条款,将会在页面公布。
如果不接受所改动的内容,用户可以主动取消自己的会员资格。如果您不取消自己的会员资格,则视为接受服务条款的变动。
4.用户的帐号、密码和安全性
一旦成功注册成为会员,您将有一个密码和用户名。
用户将对用户名和密码的安全负全部责任。另外,每个用户都要对以其用户名进行的所有活动和事件负全责。您可以随时改变您的密码。
用户若发现任何非法使用用户帐号或存在安全漏洞的情况,请立即通告本公司。
5.拒绝提供担保
用户明确同意使用本公司服务,由用户个人承担风险。
本网站及APP不担保服务一定满足用户的要求,也不担保服务不会中断,对服务的及时性、安全性、出错发生都不作担保。
用户理解并接受:任何通过服务取得的信息资料的可靠性有用性取决于用户自己的判断,用户自己承担所有风险和责任。
6.有限责任
本网站及APP对任何由于使用服务引发的直接、间接、偶然及继起的损害不负责任。
这些损害可能来自(包括但不限于):不正当使用服务,或传送的信息不符合规定等。
7.对用户信息的存储和限制
本网站及APP不对用户发布信息的删除或储存失败负责,本公司有判定用户的行为是否符合服务条款的要求和精神的保留权利。如果用户违背了服务条款的规定,有中断对其提供服务的权利。
8.结束服务
本网站及APP可随时根据实际情况中断一项或多项服务,不需对任何个人或第三方负责或知会。
同时用户若反对任何服务条款的建议或对后来的条款修改有异议,或对服务不满,用户可以行使如下权利:
- 不再使用本公司的服务。
- 通知本公司停止对该用户的服务。
9.信息内容的所有权
本公司的信息内容包括:文字、软件、声音、相片、录象、图表;以及其它信息,所有这些内容受版权、商标、标签和其它财产所有权法律的保护。
用户只能在授权下才能使用这些内容,而不能擅自复制、再造这些内容、或创造与内容有关的派生产品。
10.隐私保护条款
本网站及APP将严格保守用户的个人隐私,承诺未经过您的同意不将您的个人信息任意披露。
但在以下情形下,我们将无法再提供您前述保证而披露您的相关信息。这些情形包括但不限于:
- 为了您的交易顺利完成,我们不得不把您的某些信息,如您的姓名、联系电话、e-mail等提供给相关如物流服务方,以便于他们及时与您取得联系,提供服务。
- 当您在本网站的行为违反的服务条款,或可能损害他人权益或导致他人遭受损害,只要我们相信披露您的个人资料是为了辨识、联络或采取法律行动所必要的行动时。
- 法律法规所规定的必须披露或公开的个人信息。
- 当司法机关或其它有权机关依法执行公务,要求提供特定个人资料时,我们必须给予必要的配合。
11.适用法律
上述条款将适用中华人民共和国的法律,所有的争端将诉诸于本网所在地的人民法院。
如发生服务条款与中华人民共和国法律相抵触时,则这些条款将完全按法律规定重新解释,而其它条款则依旧保持约束力。
<file_sep>/app/authx/authx/health.sh
ps -elf |grep authx |grep -v grep
<file_sep>/single/manuals.md
K2Fuxi
======
使用说明
----
本应用分为检索端和数据管理端。
检索端提供数据的搜索、统计,数据管理端提供数据的导入。
##### 检索端web访问
----
Web登录:http://{K2Fuxi Service IP}:8080
用户名:admin
密码:<PASSWORD>
----
##### 数据管理端Web访问
----
Web登录:http://{K2Fuxi Service IP}:8081
用户名:admin
密码:<PASSWORD>
数据管理端提供工单管理、词库管理、Tag管理。
----
##### 示例操作
1. 下载工单xlsx文件 https://github.com/Tsui89/qcloud/blob/new-k2fuxi/single/FA2016.xlsx
2. 登陆数据管理端Web,在工单导入界面导入下载的工单文件。
3. 登陆检索端web,工单搜索故障
<file_sep>/app/authx/authx/stop.sh
#!/bin/bash
ps -ef |grep authx |grep -v 'grep' |awk -F ' ' '{print $2}' |xargs kill -9
<file_sep>/app/authx/authx/start.sh
#!/bin/bash
/opt/authx/authx /opt/authx/config.yaml &
<file_sep>/app/authx/confd/conf.d/authx.config.toml
[template]
src = "authx.config.tmpl"
dest = "/opt/authx/config.yaml"
keys = [
"/",
]
reload_cmd = "/opt/authx/restart.sh"
| 8991ad7bba3eba8918a8754d6f779aa7d2a73150 | [
"Markdown",
"TOML",
"Python",
"Shell"
] | 9 | Shell | Tsui89/qcloud | b6c974267b4a94aa0f8bae1a2af7ee9f8f11bcf2 | 0c575170211fbf8a99408864d1e455fdeab5b174 | |
refs/heads/master | <repo_name>Natt7/JINSHANG<file_sep>/complete/JINSHANG-110/schema.sql
create database db;
use db;
create schema T_GG_SJ_GOODS_schema
source type CSV
fields(
"goodsid" type string,
"deptid" type string,
"packnum" type string,
"barcode" type string,
"goodslocid" type string,
"stocktype" type string,
"ownerid" type string,
"ownername" type string,
"goodsownermanageno" type string,
"inbillid" type string,
"goodsownerpackno" type string,
"manageno" type string,
"feebegindate" type DATETIME,
"contractid" type string,
"goodsstateno" type string,
"goodsstatename" type string,
"changeownertimes" type double default value '0',
"goodstype" type string,
"goodstypename" type string,
"kindno" type string,
"kindname" type string,
"shopsignid" type string,
"shopsignname" type string,
"prodareaid" type string,
"prodareaname" type string,
"goodsbatchno" type string,
"prodstoveno" type string,
"gradenum" type string,
"gradenumthick" type double,
"gradenumwidth" type double,
"gradenumlength" type double,
"grossweight" type double,
"netweight" type double,
"poundweight" type double,
"checkweight" type double,
"sheetnum" type double default value '1',
"unit" type string,
"packtype" type string,
"stockareaid" type string,
"qualitymemo" type string,
"firstinownerid" type string,
"firstindate" type DATETIME,
"outbillid" type string,
"ldglistid" type string,
"materialgoodsid" type string,
"intype" type string,
"outtype" type string,
"billstate" type double,
"outbilltime" type DATETIME,
"memo" type string,
"extsysplanobjectid" type string,
"extsyskindid" type string,
"extsysshopsignid" type string,
"extsysprodareaid" type string,
"foreignlistno" type string,
"sendflag" type double,
"r_record_create_date" type DATETIME,
"r_record_create_user" type string,
"r_record_update_date" type DATETIME,
"r_record_update_user" type string,
"r_record_is_deleted" type double,
"incusttime" type DATETIME,
"sidemark" type string,
"temp" type string,
"length_product_max" type double,
"heat_num" type string,
"test_lot_num" type string,
"r_record_change_flag" type double,
"sdprice" type string,
"extsysgradenum" type string,
"collectionmanname" type string,
"lockflag" type string,
"check_type" type string,
"outdate" type DATETIME,
"yfweight" type double,
"yfsheet" type double,
"pregoodsid" type string,
"issplit" type double,
"gradenumxh" type double,
"gradenumwhdw" type string,
"splittime" type double,
"isclear" type double,
"singlesheetnum" type double,
"gradenumhz" type string,
"gradenumthickhz" type double,
"singlesheet" type double,
"loclv1" type string,
"singlesheetunit" type string,
"dcemp" type string,
"qzemp" type string,
"dcemp2" type string,
"shipno" type string,
"shipname" type string,
"shipcd" type string,
"shiplevel" type string,
"cmmemo" type string,
"cmmemock" type string,
"shopsigncd" type string,
"processtypename" type string,
"packtypename" type string,
"sort_emp" type string,
"customerid" type string,
"lvgoodsid" type string,
"dispersesheet" type double,
"dbdeptid" type string,
"dbcustomer" type string,
"dbgulender" type string,
"locked_object" type string,
"locked_date" type DATETIME,
"unlocked_date" type DATETIME,
"preownername" type string,
"ply" type double default value '0',
"tdstate" type string default value '1',
"opselected" type string,
"oplock" type string,
"opinupload" type string,
"opchangeupload" type string,
"opinbatchid" type string,
"opchangebatchid" type string,
"opinupload_date" type DATETIME,
"out_package_status" type string,
"out_package_desc" type string,
"gmpacktype" type string,
"handno" type string,
"saveno" type string,
"drawno" type string,
"dangerouslevel" type string,
"acceptancecriteria" type string,
"shippingmark" type string,
"loclv2" type string,
"payedflag" type string,
"ordergoodsownerid" type string,
"ordergoodsownername" type string
)
RECORD DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_GG_SJ_GOODS_parser
TYPE rcd
SCHEMA T_GG_SJ_GOODS_schema;
CREATE TABLE T_GG_SJ_GOODS using T_GG_SJ_GOODS_parser;
create schema T_ZY_RK_INBILL_schema
SOURCE TYPE CSV
FIELDS(
"inbillid" type string,
"deptid" type string,
"foreignlistno" type string,
"goodsbatchno" type string,
"intype" type string,
"feebegindate" type DATETIME,
"incusttime" type DATETIME,
"inbillownername" type string,
"inbillownerno" type string,
"inbillempno" type string,
"inbillempname" type string,
"inbilltime" type DATETIME,
"inbillmemo" type string,
"kindno" type string,
"kindname" type string,
"shopsignname" type string,
"prodareaname" type string,
"prodareaid" type string,
"remanageno" type string,
"shopsignid" type string,
"truckgroupname" type string,
"truckgroupno" type string,
"planno" type string,
"manageno" type string,
"sheetnumsum" type double,
"grossweightsum" type double,
"netweightsum" type double,
"goodsownermanageno" type string,
"firstindate" type DATETIME,
"gradenum" type string,
"r_record_create_date" type DATETIME,
"r_record_create_user" type string,
"r_record_update_date" type DATETIME,
"r_record_update_user" type string,
"r_record_is_deleted" type double,
"stocktype" type string,
"r_record_change_flag" type double,
"rktype" type string,
"trans_type" type string,
"finish_date" type DATETIME,
"check_date" type DATETIME,
"check_type" type string,
"sort_emp" type string,
"check_emp" type string,
"loclv1" type string,
"loclv2" type string,
"dcemp" type string,
"qzemp" type string,
"dcemp2" type string,
"sendmessage" type string,
"dtlcount" type double,
"sidemark" type string,
"ischecked" type double,
"sheetsumsum" type double,
"issettled" type double,
"handno" type string,
"saveno" type string,
"deptfrom" type string,
"jfsendflag" type double,
"checkdtlcount" type double
)
SCHEMA DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_ZY_RK_INBILL_parser
TYPE rcd
SCHEMA T_ZY_RK_INBILL_schema;
CREATE TABLE T_ZY_RK_INBILL using T_ZY_RK_INBILL_parser;
CREATE SCHEMA T_ZY_CK_OUTBILL_schema
SOURCE TYPE CSV
FIELDS(
"outbillid" type string,
"deptid" type string,
"foreignlistno" type string,
"outbillownername" type string,
"outbillownerno" type string,
"outbillpickownername" type string,
"outbillpickownerno" type string,
"outtype" type string,
"outcusttime" type datetime,
"outbillempno" type string,
"outbillempname" type string,
"outbilltime" type datetime,
"newmanagenum" type string,
"truckgroupno" type string,
"outcarno" type string,
"outbillmemo" type string,
"planno" type string,
"netweightsum" type double,
"sheetnumsum" type double,
"r_record_create_date" type datetime,
"r_record_create_user" type string,
"r_record_update_date" type datetime,
"r_record_update_user" type string,
"r_record_is_deleted" type double,
"outstate" type string,
"invoicekindid" type string,
"invoicekindname" type string,
"r_record_change_flag" type double,
"printcount" type double,
"maxprintcount" type double,
"issplit" type double,
"outtypename" type string,
"check_type" type string,
"operator" type string,
"outcardrivername" type string,
"idcode" type string,
"settlenum" type string,
"outpassword" type string,
"sendmessage" type string,
"dtlcount" type double,
"confirm_flag" type string default value '0',
"confirm_date" type datetime,
"confirm_emp" type string,
"issettled" type double,
"deptto" type string,
"trans_type" type string,
"jfsendflag" type double,
"jyzxflag" type double,
"username" type string,
"billlock" type string
)
SCHEMA DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_ZY_CK_OUTBILL_parser
TYPE rcd
SCHEMA T_ZY_CK_OUTBILL_schema;
CREATE TABLE T_ZY_CK_OUTBILL using T_ZY_CK_OUTBILL_parser;
create schema T_ZY_JG_PROCESS_schema
SOURCE TYPE CSV
FIELDS(
"processid" type string,
"deptid" type string,
"customerid" type string,
"processdept" type string,
"processdeptname" type string,
"processtype" type string,
"processtypename" type string,
"processmold" type string,
"processmoldname" type string,
"processstate" type double,
"planenddate" type datetime,
"kindno" type string,
"kindname" type string,
"packtype" type string,
"packtypename" type string,
"woodtype" type string,
"woodtypename" type string,
"straptype" type string,
"straptypename" type string,
"processprecision" type string,
"orderinfo" type string,
"fee" type double,
"length" type double,
"weigtht" type double,
"sheet" type double,
"piecenum" type double,
"outcarbilltime" type datetime,
"outcarbillempno" type string,
"outcarbillempname" type string,
"planno" type string,
"preweightsum" type double,
"meno" type string,
"gradenumrange" type string,
"gradenumrangename" type string,
"thickrange" type string,
"thickrangename" type string,
"r_record_create_date" type datetime,
"r_record_create_user" type string,
"r_record_update_date" type datetime,
"r_record_update_user" type string,
"r_record_is_deleted" type double,
"r_record_change_flag" type double,
"density" type double,
"processcustomno" type string,
"processcustomname" type string,
"settledeptno" type string,
"settledeptname" type string,
"settle_staus" type string,
"settle_staus_name" type string,
"settle_no" type string,
"settle_date" type datetime,
"acceptancer" type string,
"acceptance_time" type datetime,
"chanxian" type string,
"mddeptname" type string,
"articleys" type string,
"materialgradenum" type string,
"materialshopsign" type string,
"cbxjgtype" type string,
"jgmessage" type string,
"jgstatus" type string,
"articlefee" type string,
"issettled" type double,
"specialdown" type string,
"articlesheet" type double,
"articleweight" type double,
"username" type string,
"jfsendflag" type double,
"isreceived" type string,
"specialmemo" type string,
"toprocessid" type string,
"jxlocked" type string
)
SCHEMA DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_ZY_JG_PROCESS_parser
TYPE rcd
SCHEMA T_ZY_JG_PROCESS_schema;
CREATE TABLE T_ZY_JG_PROCESS using T_ZY_JG_PROCESS_parser;
create schema union_all_schema
source type csv
fields(
"store_amount" type double,
"store_in_amount" type double,
"store_out_amount" type double,
"store_process_amount" type double
)
schema delimiter "LF"
field delimiter ","
text qualifier "DQM";
create parser union_all_parser
type rcd
schema union_all_schema;
create table union_all using union_all_parser;
create index union_all_index on table union_all(store_amount);
create statistics model union_all_model on table union_all
measures (sum(store_amount),sum(store_in_amount),sum(store_out_amount),sum(store_process_amount));
<file_sep>/schema/T_SS_SMS_SEND_LOG.sql
create schema T_SS_SMS_SEND_LOG_schema
SOURCE TYPE CSV
FIELDS(
"seg_no" type str,
"send_index" type str,
"send_id" type str,
"important_flag" type str,
"priority" type str,
"customer_id" type str,
"reciever_name" type str,
"destination_mobile" type str,
"sms_message" type str,
"sender_sign" type str,
"remark" type str,
"operator" type str,
"plan_send_date" type DATETIME,
"operate_date" type DATETIME,
"send_date" type DATETIME,
"business_no" type str,
"module_code" type str,
"deptid" type str
)
SCHEMA DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_SS_SMS_SEND_LOG_parser
TYPE rcd
SCHEMA T_SS_SMS_SEND_LOG_schema;
CREATE TABLE T_SS_SMS_SEND_LOG using T_SS_SMS_SEND_LOG_parser;
create index T_SS_SMS_SEND_LOG_index on table T_SS_SMS_SEND_LOG(seg_no);<file_sep>/complete/JINSHANG-110/del_4.sql
create job del_4_job(2)
begin
dataset file T_ZY_JG_PROCESS_dataset_22
(
schema: T_ZY_JG_PROCESS_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_jg_process/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc select union_select
(
inputs: "T_ZY_JG_PROCESS_dataset_22",
fields:
(
(fname:"0",alias:"store_amount",type:"double"),
(fname:"0",alias:"store_in_amount",type:"double"),
(fname:"0",alias:"store_out_amount",type:"double"),
(fname:"preweightsum",alias:"store_process_amount",type:"double")
),
distinct: true,
data_opr:delete,
cache:true,
conditions:" deptid NOT LIKE '03%'
AND deptid NOT LIKE 'ZZ%'
AND deptid NOT LIKE 'MG%' "
);
dataproc statistics T_ZY_JG_PROCESS_del_statistics
(
inputs:union_select,
table:union_all,
data_opr:delete
);
end;
run job del_4_job (threads:8);<file_sep>/uncomplete/JINSHANG-94/jinshang-94.sql
INSERT INTO SHOW.PDGM_SHOWKC --库存量的数据来源
SELECT PROVIDER_NAME,
sum(NETWEIGHTSUM),
sum(KCTDNET),
0
FROM
( SELECT PROVIDER_NAME,
NETWEIGHTSUM,
0 KCTDNET
FROM
( SELECT '广东韶钢松山股份有限公司' PROVIDER_NAME,
0 NETWEIGHTSUM
FROM dual
UNION ALL --梅钢
SELECT '上海梅山钢铁股份有限公司' PROVIDER_NAME,
sum(netweight) NETWEIGHTSUM
FROM wmsdba.t_gg_sj_goods t
WHERE goodsstateno=20
AND EXISTS
(SELECT 1
FROM WL.T_OWNER W
WHERE SEG_NO='00103'
AND T.DEPTID=W.DEPTID
AND t.ownerid=w.owner_id
AND (w.deptid!='0301'
AND w.deptid!='4501'))
AND EXISTS
(SELECT 1
FROM wl.t_his_t_stacking_m m,wl.t_his_t_stacking_d d
WHERE m.manu_id=d.manu_id
AND m.stacking_rec_num=d.stacking_rec_num
AND m.seg_no='00103'
AND m.manu_id='BGTM'
AND t.packnum=d.pack_num)
UNION ALL --股份
SELECT '宝山钢铁股份有限公司' PROVIDER_NAME,
sum(netweight) NETWEIGHTSUM
FROM wmsdba.t_gg_sj_goods t
WHERE goodsstateno=20
AND EXISTS
(SELECT 1
FROM WL.T_OWNER W
WHERE SEG_NO='00103'
AND T.DEPTID=W.DEPTID
AND t.ownerid=w.owner_id
AND (w.deptid!='0301'
AND w.deptid!='4501'))
AND EXISTS
(SELECT 1
FROM wl.t_his_t_stacking_m m,wl.t_his_t_stacking_d d
WHERE m.manu_id=d.manu_id
AND m.stacking_rec_num=d.stacking_rec_num
AND m.seg_no='00103'
AND m.manu_id='BGSA'
AND t.packnum=d.pack_num)
UNION ALL --特殊钢
SELECT '宝钢特钢有限公司' PROVIDER_NAME,
sum(netweight) NETWEIGHTSUM
FROM wmsdba.t_gg_sj_goods t
WHERE goodsstateno=20
AND EXISTS
(SELECT 1
FROM WL.T_OWNER W
WHERE SEG_NO='00103'
AND T.DEPTID=W.DEPTID
AND t.ownerid=w.owner_id
AND (w.deptid!='0301'
AND w.deptid!='4501'))
AND EXISTS
(SELECT 1
FROM wl.t_his_t_stacking_m m,wl.t_his_t_stacking_d d
WHERE m.manu_id=d.manu_id
AND m.stacking_rec_num=d.stacking_rec_num
AND m.seg_no='00103'
AND m.manu_id='BGSW'
AND t.packnum=d.pack_num))
UNION ALL --已开单
SELECT PROVIDER_NAME,
0,
NETWEIGHTSUM
FROM
( SELECT '广东韶钢松山股份有限公司' PROVIDER_NAME,
0 NETWEIGHTSUM
FROM dual
UNION ALL --梅钢
SELECT '上海梅山钢铁股份有限公司' PROVIDER_NAME,
sum(netweight) NETWEIGHTSUM
FROM wmsdba.t_gg_sj_goods t
WHERE goodsstateno=20
AND EXISTS
(SELECT 1
FROM WL.T_OWNER W
WHERE SEG_NO='00103'
AND T.DEPTID=W.DEPTID
AND t.ownerid=w.owner_id
AND (w.deptid!='0301'
AND w.deptid!='4501'))
AND EXISTS
(SELECT 1
FROM wl.t_his_t_stacking_m m,wl.t_his_t_stacking_d d
WHERE m.manu_id=d.manu_id
AND m.stacking_rec_num=d.stacking_rec_num
AND m.seg_no='00103'
AND m.manu_id='BGTM'
AND t.packnum=d.pack_num)
AND exists
(SELECT 1
FROM wl.t_s_bill_d d
WHERE t.packnum=d.pack_id
AND d.seg_no='00103')
UNION ALL --股份
SELECT '宝山钢铁股份有限公司' PROVIDER_NAME,
sum(netweight) NETWEIGHTSUM
FROM wmsdba.t_gg_sj_goods t
WHERE goodsstateno=20
AND EXISTS
(SELECT 1
FROM WL.T_OWNER W
WHERE SEG_NO='00103'
AND T.DEPTID=W.DEPTID
AND t.ownerid=w.owner_id
AND (w.deptid!='0301'
AND w.deptid!='4501'))
AND EXISTS
(SELECT 1
FROM wl.t_his_t_stacking_m m,wl.t_his_t_stacking_d d
WHERE m.manu_id=d.manu_id
AND m.stacking_rec_num=d.stacking_rec_num
AND m.seg_no='00103'
AND m.manu_id='BGSA'
AND t.packnum=d.pack_num)
AND exists
(SELECT 1
FROM wl.t_s_bill_d d
WHERE t.packnum=d.pack_id
AND d.seg_no='00103')
UNION ALL --特殊钢
SELECT '宝钢特钢有限公司' PROVIDER_NAME,
sum(netweight) NETWEIGHTSUM
FROM wmsdba.t_gg_sj_goods t
WHERE goodsstateno=20
AND EXISTS
(SELECT 1
FROM WL.T_OWNER W
WHERE SEG_NO='00103'
AND T.DEPTID=W.DEPTID
AND t.ownerid=w.owner_id
AND (w.deptid!='0301'
AND w.deptid!='4501'))
AND EXISTS
(SELECT 1
FROM wl.t_his_t_stacking_m m,wl.t_his_t_stacking_d d
WHERE m.manu_id=d.manu_id
AND m.stacking_rec_num=d.stacking_rec_num
AND m.seg_no='00103'
AND m.manu_id='BGSW'
AND t.packnum=d.pack_num)
AND exists
(SELECT 1
FROM wl.t_s_bill_d d
WHERE t.packnum=d.pack_id
AND d.seg_no='00103')) )
GROUP BY PROVIDER_NAME;
<file_sep>/complete/JINSHANG-110/init.sql
create job 1_job(2)
begin
dataset file T_GG_SJ_GOODS_dataset
(
schema: T_GG_SJ_GOODS_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_GG_SJ_GOODS.csv",
serverid: 0,
charset:utf-8,
splitter: (block_size: 10000000)
);
dataset file T_ZY_RK_INBILL_dataset
(
schema: T_ZY_RK_INBILL_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_RK_INBILL.csv",
serverid: 0,
charset:utf-8,
splitter: (block_size: 10000000)
);
dataset file T_ZY_CK_OUTBILL_dataset
(
schema: T_ZY_CK_OUTBILL_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_CK_OUTBILL.csv",
serverid: 0,
charset:utf-8,
splitter: (block_size: 10000000)
);
dataset file T_ZY_JG_PROCESS_dataset
(
schema: T_ZY_JG_PROCESS_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_JG_PROCESS.csv",
serverid: 0,
charset:utf-8,
splitter: (block_size: 10000000)
);
dataproc select 1_select
(
fields:
(
(fname:"netweight",alias:"store_amount",type:"double"),
(fname:"0",alias:"store_in_amount",type:"double"),
(fname:"0",alias:"store_out_amount",type:"double"),
(fname:"0",alias:"store_process_amount",type:"double")
),
inputs: "T_GG_SJ_GOODS_dataset",
order_by:("store_process_amount"),
conditions:" goodsstateno = '110'
AND deptid NOT LIKE '03%'
AND deptid NOT LIKE 'ZZ%'
AND deptid NOT LIKE 'MG%' "
);
dataproc select 2_select
(
fields:
(
(fname:"0",alias:"store_amount",type:"double"),
(fname:"netweightsum",alias:"store_in_amount",type:"double"),
(fname:"0",alias:"store_out_amount",type:"double"),
(fname:"0",alias:"store_process_amount",type:"double")
),
inputs: "T_ZY_RK_INBILL_dataset",
order_by:("store_process_amount"),
conditions:" deptid NOT LIKE '03%'
AND deptid NOT LIKE 'ZZ%'
AND deptid NOT LIKE 'MG%' "
);
dataproc select 3_select
(
fields:
(
(fname:"0",alias:"store_amount",type:"double"),
(fname:"0",alias:"store_in_amount",type:"double"),
(fname:"netweightsum",alias:"store_out_amount",type:"double"),
(fname:"0",alias:"store_process_amount",type:"double")
),
inputs: "T_ZY_CK_OUTBILL_dataset",
order_by:("store_process_amount"),
conditions:" deptid NOT LIKE '03%'
AND deptid NOT LIKE 'ZZ%'
AND deptid NOT LIKE 'MG%' "
);
dataproc select 4_select
(
fields:
(
(fname:"0",alias:"store_amount",type:"double"),
(fname:"0",alias:"store_in_amount",type:"double"),
(fname:"0",alias:"store_out_amount",type:"double"),
(fname:"preweightsum",alias:"store_process_amount",type:"double")
),
inputs: "T_ZY_JG_PROCESS_dataset",
order_by:("store_process_amount"),
conditions:" deptid NOT LIKE '03%'
AND deptid NOT LIKE 'ZZ%'
AND deptid NOT LIKE 'MG%' "
);
dataproc union 1_union
(
inputs:("1_select","2_select","3_select","4_select")
);
dataproc select union_select
(
fields:
(
(fname:"store_amount",type:"double"),
(fname:"store_in_amount",type:"double"),
(fname:"store_out_amount",type:"double"),
(fname:"store_process_amount",type:"double")
),
inputs:"1_union",
cache:true
);
dataproc statistics union_all_statistics
(
inputs:union_select,
table:union_all
);
end;
run job 1_job (threads:8);
<file_sep>/uncomplete/JINSHANG-74/jinshang-74.sql
SELECT *
FROM
(SELECT rownum AS rownum_,
aa.*
FROM
(SELECT nvl(a.manageno,'null') AS MANAGENO,
nvl(a.kindname,'null') AS KINDNAME,
nvl(a.shopsignname,'null') AS SHOPSIGNNAME,
nvl(a.prodareaname,'null') AS PRODAREANAME,
sum(decode(a.goodtype, '10', a.netweight, 0)) AS INSTOCK,
sum(decode(a.goodtype, '20', a.netweight, 0)) AS OUTSTOCK,
sum(decode(a.goodtype, '11', a.netweight, 0)) AS CHANGINSTOCK ,
sum(decode(a.goodtype, '21', a.netweight, 0)) AS CHANGOUTSTOCK,
sum(decode(a.goodtype, '12', a.netweight, 0)) AS PROCESSINSTOCK,
sum(decode(a.goodtype, '22', a.netweight, 0)) AS PROCESSOUTSTOCK,
sum(decode(a.goodtype, '30', a.netweight, 0)) AS ENDSTOCK,
sum(decode(a.goodtype, '30', a.netweight, 0)) - sum(decode(a.goodtype, '10', a.netweight, 0)) + sum(decode(a.goodtype, '20', a.netweight, 0)) AS BEGINSTOCK
FROM
(SELECT t1.manageno,
t1.kindname,
t1.shopsignname,
t1.gradenum,
t1.prodareaname,
t1.deptid,
'10' goodtype,
t1.netweight
FROM wmsdba.t_zy_rk_inbilldetail t1 ,
ST.T_XTSZ_BIND b
WHERE to_char(t1.firstindate, 'yyyy-mm-dd') >= '2015-07-16'
AND to_char(t1.firstindate, 'yyyy-mm-dd') <= '2015-07-16'
AND t1.deptid=b.dept_id
AND t1.ownerid=b.owner_id
AND b.alive_flag='1'
AND b.seg_no='080001'
UNION ALL SELECT t2.managenum,
t2.kindname,
t2.shopsignname,
t2.gradenum,
t2.prodareaname,
t2.deptid,
'20',
t2.netweight
FROM wmsdba.t_zy_ck_outpack t2,
wmsdba.t_zy_ck_outbill t3 ,
ST.T_XTSZ_BIND b
WHERE t2.deptid = t3.deptid
AND t2.outbillno = t3.outbillid
AND to_char(t2.OutCustTime, 'yyyy-mm-dd') >= '2015-07-16'
AND to_char(t2.OutCustTime, 'yyyy-mm-dd') <= '2015-07-16'
AND t2.deptid=b.dept_id
AND t2.ownerno=b.owner_id
AND b.alive_flag='1'
AND b.seg_no='080001'
UNION ALL SELECT t4.managenum,
t4.kindname,
t4.shopsignname,
t4.gradenum,
t4.prodareaname,
t4.deptid,
'11',
t4.netweight
FROM wmsdba.t_zy_gh_changeownerdetail t4,
wmsdba.t_zy_gh_changeownerlist t5 ,
ST.T_XTSZ_BIND b
WHERE t4.deptid = t5.deptid
AND t4.changeownerno = t5.changeownerid
AND to_char(t5.changedate, 'yyyy-mm-dd') >= '2015-07-16'
AND to_char(t5.changedate, 'yyyy-mm-dd') <= '2015-07-16'
AND t4.deptid=b.dept_id
AND t5.newownerno=b.owner_id
AND b.alive_flag='1'
AND b.seg_no='080001'
UNION ALL SELECT t4.managenum,
t4.kindname,
t4.shopsignname,
t4.gradenum,
t4.prodareaname,
t4.deptid,
'21',
t4.netweight
FROM wmsdba.t_zy_gh_changeownerdetail t4,
wmsdba.t_zy_gh_changeownerlist t5 ,
ST.T_XTSZ_BIND b
WHERE t4.deptid = t5.deptid
AND t4.changeownerno = t5.changeownerid
AND to_char(t5.changedate, 'yyyy-mm-dd') >= '2015-07-16'
AND to_char(t5.changedate, 'yyyy-mm-dd') <= '2015-07-16'
AND t4.deptid=b.dept_id
AND t5.preownerno=b.owner_id
AND b.alive_flag='1'
AND b.seg_no='080001'
UNION ALL SELECT t6.manageno,
t6.kindname,
t6.shopsignname,
t6.gradenum,
t6.prodareaname,
t6.deptid,
'12',
t6.netweight
FROM wmsdba.t_zy_jg_procarticledtl t6 ,
ST.T_XTSZ_BIND b
WHERE t6.deptid LIKE '080001'||'%'
AND to_char(t6.ACCEPTANCE_TIME, 'yyyy-mm-dd') >= '2015-07-16'
AND to_char(t6.acceptance_time, 'yyyy-mm-dd') <= '2015-07-16'
AND t6.deptid=b.dept_id
AND t6.ownerid=b.owner_id
AND b.alive_flag='1'
AND b.seg_no='080001'
UNION ALL SELECT t7.manageno,
t7.kindname,
t7.shopsignname,
t7.gradenum,
t7.prodareaname,
t7.deptid,
'22',
t7.netweight
FROM wmsdba.t_zy_jg_procmaterialdtl t7,
wmsdba.t_zy_jg_process t8 ,
ST.T_XTSZ_BIND b
WHERE t7.deptid LIKE '080001'||'%'
AND t7.deptid = t8.deptid
AND t7.processid = t8.processid
AND to_char(t8.outcarbilltime, 'yyyy-mm-dd') >= '2015-07-16'
AND to_char(t8.outcarbilltime, 'yyyy-mm-dd') <= '2015-07-16'
AND t7.deptid=b.dept_id
AND t7.ownerid=b.owner_id
AND b.alive_flag='1'
AND b.seg_no='080001'
UNION ALL SELECT b.manageno,
b.kindname,
b.shopsignname,
b.gradenum,
b.prodareaname,
b.deptid,
'30',
b.netweight
FROM
(SELECT /*+ index(t,T_GG_SJ_GOODS_INDX02) */ t.manageno,
t.kindname,
t.shopsignname,
t.gradenum,
t.prodareaname,
t.deptid,
t.netweight
FROM wmsdba.t_gg_sj_goods t ,
ST.T_XTSZ_BIND b
WHERE 1=1
AND t.goodsstateno = '20'
AND t.deptid=b.dept_id
AND t.ownerid=b.owner_id
AND b.alive_flag='1'
AND b.seg_no='080001'
UNION ALL SELECT t1.manageno,
t1.kindname,
t1.shopsignname,
t1.gradenum,
t1.prodareaname,
t1.deptid,
0 - t1.netweight
FROM wmsdba.t_zy_rk_inbilldetail t1 ,
ST.T_XTSZ_BIND b
WHERE to_char(t1.firstindate, 'yyyy-mm-dd') > '2015-07-16'
AND t1.deptid=b.dept_id
AND t1.ownerid=b.owner_id
AND b.alive_flag='1'
AND b.seg_no='080001'
UNION ALL SELECT t4.managenum,
t4.kindname,
t4.shopsignname,
t4.gradenum,
t4.prodareaname,
t4.deptid,
0 - t4.netweight
FROM wmsdba.t_zy_gh_changeownerdetail t4,
wmsdba.t_zy_gh_changeownerlist t5 ,
ST.T_XTSZ_BIND b
WHERE t4.deptid = t5.deptid
AND t4.changeownerno = t5.changeownerid
AND to_char(t5.changedate, 'yyyy-mm-dd') > '2015-07-16'
AND t4.deptid=b.dept_id
AND t5.newownerno=b.owner_id
AND b.alive_flag='1'
AND b.seg_no='080001'
UNION ALL SELECT t6.manageno,
t6.kindname,
t6.shopsignname,
t6.gradenum,
t6.prodareaname,
t6.deptid,
0 - t6.netweight
FROM wmsdba.t_zy_jg_procarticledtl t6 ,
ST.T_XTSZ_BIND b
WHERE t6.deptid LIKE '080001'||'%'
AND to_char(t6.ACCEPTANCE_TIME, 'yyyy-mm-dd') > '2015-07-16'
AND t6.deptid=b.dept_id
AND t6.ownerid=b.owner_id
AND b.alive_flag='1'
AND b.seg_no='080001'
UNION ALL SELECT t2.managenum,
t2.kindname,
t2.shopsignname,
t2.gradenum,
t2.prodareaname,
t2.deptid,
t2.netweight
FROM wmsdba.t_zy_ck_outpack t2,
wmsdba.t_zy_ck_outbill t3 ,
ST.T_XTSZ_BIND b
WHERE t2.deptid = t3.deptid
AND t2.outbillno = t3.outbillid
AND to_char(t3.outbilltime, 'yyyy-mm-dd') > '2015-07-16'
AND t2.deptid=b.dept_id
AND t2.ownerno=b.owner_id
AND b.alive_flag='1'
AND b.seg_no='080001'
UNION ALL SELECT t4.managenum,
t4.kindname,
t4.shopsignname,
t4.gradenum,
t4.prodareaname,
t4.deptid,
t4.netweight
FROM wmsdba.t_zy_gh_changeownerdetail t4,
wmsdba.t_zy_gh_changeownerlist t5 ,
ST.T_XTSZ_BIND b
WHERE t4.deptid = t5.deptid
AND t4.changeownerno = t5.changeownerid
AND to_char(t5.changedate, 'yyyy-mm-dd') > '2015-07-16'
AND t4.deptid=b.dept_id
AND t5.preownerno=b.owner_id
AND b.alive_flag='1'
AND b.seg_no='080001'
UNION ALL SELECT t7.manageno,
t7.kindname,
t7.shopsignname,
t7.gradenum,
t7.prodareaname,
t7.deptid,
t7.netweight
FROM wmsdba.t_zy_jg_procmaterialdtl t7,
wmsdba.t_zy_jg_process t8 ,
ST.T_XTSZ_BIND b
WHERE t7.deptid LIKE '080001'||'%'
AND t7.deptid = t8.deptid
AND t7.processid = t8.processid
AND to_char(t8.outcarbilltime, 'yyyy-mm-dd') > '2015-07-16'
AND t7.deptid=b.dept_id
AND t7.ownerid=b.owner_id
AND b.alive_flag='1'
AND b.seg_no='080001') b) a
GROUP BY a.manageno,
a.kindname,
a.shopsignname,
a.prodareaname)aa)
WHERE rownum_ > 1
AND rownum_ <= 10<file_sep>/uncomplete/JINSHANG-100/jinshang-100.sql
SELECT *
FROM
(SELECT gg.ownerid,
'' ownername,
gg.gradenumxh,
gg.singlesheetnum,
gg.SideMark,
gg.ShipName,
gg.netweight,
gg.grossweight,
gg.sheetnum,
gl.PACKNUM,
gl.MANAGENO,
gl.GRADENUM,
gl.GOODSLOCID,
gl.KINDNAME,
gl.SHOPSIGNNAME,
gl.PRODAREANAME,
gl.FIRSTINDATE
FROM
( SELECT pregoodsid,
tbx.ownerid,
sum(tbx.gradenumxh) gradenumxh,
sum(tbx.singlesheetnum) singlesheetnum,
max(SideMark) SideMark,
max(ShipName) ShipName ,
sum(tbx.netweight) netweight,
sum(tbx.grossweight) grossweight,
sum(tbx.sheetnum) sheetnum
FROM
( SELECT GOODSID,
OWNERID,
OWNERNAME,
gradenumxh,
singlesheetnum,
netweight,
grossweight,
sheetnum
FROM T_GG_SJ_Goods
WHERE goodsstateno='20'
AND r_record_is_deleted=0
AND deptid='0301'
UNION ALL SELECT GOODSID,OWNERID,OWNERNAME,-gradenumxh,-singlesheetnum,-netweight,-grossweight,-sheetnum
FROM T_ZY_RK_InBillDetail
WHERE deptid='0301'
AND goodsstateno='20'
AND firstindate>=to_date('2015/7/2','yyyy-mm-dd')
UNION ALL SELECT PROCARTICLEDTLID,
OWNERID,
OWNERNAME,
0 gradenumxh,
0 singlesheetnum,-netweight,-grossweight,-sheetnum
FROM T_ZY_JG_ProcArticleDtl
WHERE deptid='0301'
AND firstindate>=to_date('2015/7/2','yyyy-mm-dd')
AND goodsstateno='130'
UNION ALL SELECT GOODSID,
newownerno OWNERID,
newownername OWNERNAME,
0 gradenumxh,
0 singlesheetnum,-netweight,-grossweight,-sheetnum
FROM
(SELECT b.goodsid,
a.newownerno,
a.newownername,
b.netweight,
b.grossweight,
b.sheetnum
FROM
(SELECT deptid,
ChangeOwnerID,
newownerno,
newownername
FROM T_ZY_GH_ChangeOwnerList
WHERE DeptID='0301'
AND changedate>=to_date('2015/7/2','yyyy-mm-dd')) a ,
T_ZY_GH_ChangeOwnerDetail b
WHERE a.changeownerid=b.changeownerno
AND a.deptid=b.deptid) tb1
UNION ALL SELECT GOODSID,
OWNERNO,
OWNERNAME,
gradenumxh,
singlesheetnum,
netweight,
grossweight,
sheetnum
FROM T_ZY_CK_OutPack
WHERE deptid='0301'
AND OutCustTime>=to_date('2015/7/2','yyyy-mm-dd')
UNION ALL SELECT a.GOODSID,
OWNERID,
OWNERNAME,
gradenumxh,
singlesheetnum,
netweight,
grossweight,
sheetnum
FROM T_GG_SJ_Goods a,
T_ZY_CK_CLEAR b
WHERE a.goodsid=b.goodsid
AND a.deptid='0301'
AND CREATDATE>=to_date('2015/7/2','yyyy-mm-dd')
UNION ALL SELECT GOODSID,
OWNERID,
OWNERNAME,
0 gradenumxh,
0 singlesheetnum,
netweight,
grossweight,
sheetnum
FROM T_ZY_JG_ProcMaterialDtl
WHERE deptid='0301'
AND EXISTS
(SELECT 1
FROM T_ZY_JG_PROCESS
WHERE ProcessID=T_ZY_JG_ProcMaterialDtl.ProcessID
AND deptid=T_ZY_JG_ProcMaterialDtl.deptid
AND OutCarBillTime>=to_date('2015/7/2','yyyy-mm-dd'))
UNION ALL SELECT GOODSID,
preownerno OWNERID,
proownername OWNERNAME,
0 gradenumxh,
0 singlesheetnum,
netweight,
grossweight,
sheetnum
FROM
(SELECT b.goodsid,
a.preownerno,
a.proownername,
b.netweight,
b.grossweight,
b.sheetnum
FROM
(SELECT deptid,
ChangeOwnerID,
preownerno,
proownername
FROM T_ZY_GH_ChangeOwnerList
WHERE DeptID='0301'
AND changedate>=to_date('2015/7/2','yyyy-mm-dd')) a ,
T_ZY_GH_ChangeOwnerDetail b
WHERE a.changeownerid=b.changeownerno
AND a.deptid=b.deptid) tb1)tbx
LEFT JOIN
(SELECT *
FROM t_gg_sj_goods
WHERE deptid='0301'
AND r_record_is_deleted=0) pre ON tbx.goodsid=pre.goodsid
GROUP BY pregoodsid,
tbx.ownerid )gg
LEFT JOIN
(SELECT *
FROM t_gg_sj_goods
WHERE deptid='0301'
AND r_record_is_deleted=0) gl ON gg.pregoodsid=gl.goodsid
WHERE gg.ownerid = 'KH00001'
AND gg.netweight <> 0)
WHERE netweight <> 0
AND MANAGENO IS NOT NULL;
<file_sep>/uncomplete/JINSHANG-97/schema/T_ZY_GH_CHANGEOWNERDETAIL.sql
use db;
create schema t_zy_gh_changeownerdetail_schema
source type CSV
fields(
"manageno" type str,
"deptid" type str,
"changeownerno" type str,
"packnum" type str,
"goodsid" type str,
"inbegintime" type datetime format '%Y/%m/%d %H:%M:%S',
"barcode" type str,
"goodslocid" type str,
"ownername" type str,
"ownerno" type str,
"pregoodsownermanagenum" type str,
"goodsownermanagenum" type str,
"goodsownerpacknum" type str,
"premanagenum" type str,
"managenum" type str,
"contractno" type str,
"goodsstatename" type str,
"goodsstateno" type str,
"goodstypename" type str,
"goodstype" type str,
"kindname" type str,
"kindno" type str,
"shopsignname" type str,
"shopsignid" type str,
"prodareaname" type str,
"prodareaid" type str,
"goodsbatchnum" type str,
"gradenum" type str,
"gradenumthick" type double,
"gradenumwidth" type double,
"gradenumlength" type double,
"grossweight" type double,
"netweight" type double,
"poundweight" type double,
"checkweight" type double,
"sheetnum" type double,
"qualitymemo" type str,
"firstinownerid" type str,
"firstindate" type datetime format '%Y/%m/%d %H:%M:%S',
"inbillid" type str,
"intype" type str,
"prememo" type str,
"memo" type str,
"foreignlistno" type str,
"preforeignlistno" type str,
"orderlistno" type str,
"preorderlistno" type str,
"r_record_create_date" type datetime format '%Y/%m/%d %H:%M:%S',
"r_record_create_user" type str,
"r_record_update_date" type datetime format '%Y/%m/%d %H:%M:%S',
"r_record_update_user" type str,
"r_record_is_deleted" type double,
"preincusttime" type datetime format '%Y/%m/%d %H:%M:%S',
"incusttime" type datetime format '%Y/%m/%d %H:%M:%S',
"sendflag" type double,
"outchangesendflag" type double,
"changetimes" type double,
"originownername" type str,
"originownerno" type str,
"r_record_change_flag" type double,
"custday" type double,
"sdprice" type str,
"newdate" type datetime format '%Y/%m/%d %H:%M:%S',
"pregoodsid" type str,
"check_type" type str,
"singlesheetnum" type double,
"gradenumxh" type double,
"sidemark" type str,
"sort_emp" type str,
"dispersesheet" type double,
"ply" type double default value "0",
"originsheetnum" type double,
"originnetweight" type double,
"issplit" type double,
"gmpacktype" type str,
"preforeignlistcode" type str,
"confirmflag" type str default value "0",
"jyzxflag" type double,
"loclv1" type str,
"loclv2" type str,
"outnetweight" type double
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER t_zy_gh_changeownerdetail_parser
TYPE rcd
SCHEMA t_zy_gh_changeownerdetail_schema;
<file_sep>/uncomplete/JINSHANG-86/jinshang-86.sql
SELECT sum(netweight),COUNT *
FROM
( SELECT
(SELECT sum(t.net_weight)
FROM t_tm_stock_notice t
WHERE t.print_batch_id=im.bill_id) netweight,
bill_id
FROM t_im_blvalidatehistory im
WHERE R_RECORD_CREATE_DATE>to_date(to_char(sysdate,'yyyy-mm-dd'),'yyyy-mm-dd')
AND seg_no='STM06'
AND validatestate=11);
<file_sep>/processing/JINSHANG-76/add_3.sql
create job add_1_job(2)
begin
dataset file t_gg_sj_goods_dataset_11
(
schema: t_gg_sj_goods_schema,
filename: "/home/zhaoshun/Data/jingshang_data/T_GG_SJ_GOODS_add.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc select t_gg_sj_goods_select11
(
fields:
(
(fname:"GOODSID",type:"string"),
(fname:"DEPTID",type:"string"),
(fname:"GRADENUM",type:"string"),
(fname:"SHOPSIGNID",type:"string"),
(fname:"SHOPSIGNNAME",type:"string"),
(fname:"PRODAREAID",type:"string"),
(fname:"PRODAREANAME",type:"string"),
(fname:"KINDNO",type:"string"),
(fname:"KINDNAME",type:"string"),
(fname:"OWNERNAME",type:"string"),
(fname:"GOODSOWNERPACKNO",type:"string"),
(fname:"NETWEIGHT",type:"double"),
(fname:"GROSSWEIGHT",type:"double"),
(fname:"SHEETNUM",type:"double")
),
inputs: "t_gg_sj_goods_dataset_11",
distinct: true
);
dataproc distinct t_gg_sj_goods_dis
(
inputs:
(
left_input:"t_gg_sj_goods_select11",
right_input:"t_gg_sj_goods_select.output.cache"
),
left_fields:("GOODSID","DEPTID","GRADENUM","SHOPSIGNID"),
right_fields:("GOODSID","DEPTID","GRADENUM","SHOPSIGNID"),
leftadd:true,
rightadd:false
);
dataproc select t_gg_sj_goods_select
(
fields:
(
(fname:"GOODSID",type:"string"),
(fname:"DEPTID",type:"string"),
(fname:"GRADENUM",type:"string"),
(fname:"SHOPSIGNID",type:"string"),
(fname:"SHOPSIGNNAME",type:"string"),
(fname:"PRODAREAID",type:"string"),
(fname:"PRODAREANAME",type:"string"),
(fname:"KINDNO",type:"string"),
(fname:"KINDNAME",type:"string"),
(fname:"OWNERNAME",type:"string"),
(fname:"GOODSOWNERPACKNO",type:"string"),
(fname:"NETWEIGHT",type:"double"),
(fname:"GROSSWEIGHT",type:"double"),
(fname:"SHEETNUM",type:"double")
),
inputs: "t_gg_sj_goods_dis",
cache:true
);
dataproc statistics T_GG_SJ_GOOS_statistics
(
inputs:"t_gg_sj_goods_select",
table:t_gg_sj_goods
);
end;
run job add_1_job (threads:8);
<file_sep>/complete/JINSHANG-75/delete.sql
create job t_common_dept_delete_q1(zykie)
begin
dataset file t_common_dept2_dataset
(
schema:t_common_dept_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_common_dept/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc syncher t_common_dept_syncher
(
inputs:t_common_dept2_dataset,
database:db,
operator:delete,
table:t_common_dept
);
end;
run job t_common_dept_delete_q1(threads:8);
create job t_gg_sj_goods_delete_q1(zykie)
begin
dataset file t_gg_sj_goods2_dataset
(
schema:t_gg_sj_goods_schema,
filename:"/home/natt/syncdata/oraclesyncdata/t_gg_sj_goods/delete/delete.csv",
serverid: 0,
splitter:(block_size: 10000000)
);
dataproc syncher t_gg_sj_goods_syncher
(
inputs:t_gg_sj_goods2_dataset,
database:db,
operator:delete,
table:t_gg_sj_goods
);
end;
run job t_gg_sj_goods_delete_q1(threads:8);<file_sep>/complete/JINSHANG-75/add.sql
create job t_common_dept_insert_q1(zykie)
begin
dataset file t_common_dept1_dataset
(
schema:t_common_dept_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_common_dept/insert/insert.csv",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc syncher t_common_dept_syncher
(
inputs:t_common_dept1_dataset,
database:db,
operator:add,
table:t_common_dept
);
end;
run job t_common_dept_insert_q1(threads:8);
create job t_gg_sj_goods_insert_q1(zykie)
begin
dataset file t_gg_sj_goods1_dataset
(
schema:t_gg_sj_goods_schema,
filename:"/home/natt/syncdata/oraclesyncdata/t_gg_sj_goods/insert/insert.csv",
serverid: 0,
splitter:(block_size: 10000000)
);
dataproc syncher t_gg_sj_goods_syncher
(
inputs:t_gg_sj_goods1_dataset,
database:db,
operator:add,
table:t_gg_sj_goods
);
end;
run job t_gg_sj_goods_insert_q1(threads:8);<file_sep>/complete/JINSHANG-89&90/jinshang-89.sql
SELECT kindname,
sum(netweight)
FROM t_gg_sj_goods
WHERE goodsstateno=20
AND (deptid NOT LIKE '03%'
AND deptid NOT LIKE 'ZZ%'
AND deptid NOT LIKE 'MG%')
GROUP BY kindname;
<file_sep>/uncomplete/JINSHANG-72/2_init.sql
create job T_COMMON_DEPT_job(2)
begin
dataset file T_COMMON_DEPT_dataset
(
schema: "T_COMMON_DEPT_schema",
filename: "/home/zhaoshun/Data/jinshang_New/T_COMMON_DEPT.txt",
serverid: "0",
splitter: (block_size: 10000000)
);
dataproc index T_COMMON_DEPT_index1
(
inputs: T_COMMON_DEPT_dataset,
table: T_COMMON_DEPT
);
dataproc doc T_COMMON_DEPT_doc
(
inputs:T_COMMON_DEPT_dataset,
table:T_COMMON_DEPT
);
dataproc map T_COMMON_DEPT_map
(
inputs:T_COMMON_DEPT_dataset,
table:T_COMMON_DEPT
);
end;
run job T_COMMON_DEPT_job(threads:8);
create job T_XTSZ_BIND_job(2)
begin
dataset file T_XTSZ_BIND_dataset
(
schema: "T_XTSZ_BIND_schema",
filename: "/home/zhaoshun/Data/jinshang_New/T_XTSZ_BIND.txt",
serverid: "0",
splitter: (block_size: 10000000)
);
dataproc index T_XTSZ_BIND_index
(
inputs:T_XTSZ_BIND_dataset,
table: T_XTSZ_BIND
);
dataproc doc T_BM_UI_CONFIGURE_doc
(
inputs:T_XTSZ_BIND_dataset,
table:T_XTSZ_BIND
);
dataproc map T_XTSZ_BIND_map
(
inputs:T_XTSZ_BIND_dataset,
table:T_XTSZ_BIND
);
end;
run job T_XTSZ_BIND_job(threads:8);
create job T_GG_SJ_GOODS_job(2)
begin
dataset file T_GG_SJ_GOODS_dataset
(
schema:T_GG_SJ_GOODS_schema,
filename:"/home/zhaoshun/Data/jinshang_New/T_GG_SJ_GOODS.txt",
serverid:0,
splitter:(block_size: 10000000)
);
dataproc select T_GG_SJ_GOODS_select
(
fields:
(
(fname:"nvl (DEPTID,'NULL')",alias:"DEPTID",type:"string"),
(fname:"nvl (OWNERNAME, 'NULL')",alias:"OWNERNAME",type:"string"),
(fname:"nvl (GOODSOWNERPACKNO, 'NULL')",alias:"GOODSOWNERPACKNO",type:"string"),
(fname:"GOODSSTATENO",type:"string"),
(fname:"nvl (KINDNO, 'NULL')",alias:"KINDNO",type:"string"),
(fname:"nvl (KINDNAME, 'NULL')",alias:"KINDNAME",type:"string"),
(fname:"nvl (SHOPSIGNID, 'NULL')",alias:"SHOPSIGNID",type:"string"),
(fname:"nvl (SHOPSIGNNAME, 'NULL')",alias:"SHOPSIGNNAME",type:"string"),
(fname:"nvl (PRODAREAID, 'NULL')",alias:"PRODAREAID",type:"string"),
(fname:"nvl (PRODAREANAME, 'NULL')",alias:"PRODAREANAME",type:"string"),
(fname:"nvl (GRADENUM, 'NULL')",alias:"GRADENUM",type:"string"),
(fname:"nvl (GROSSWEIGHT, 0)",alias:"GROSSWEIGHT",type:"double"),
(fname:"nvl (NETWEIGHT, 0)",alias:"NETWEIGHT",type:"double"),
(fname:"nvl (SHEETNUM, 0)",alias:"SHEETNUM",type:"double"),
(fname:"FIRSTINDATE",type:"datetime"),
(fname:"iilmap('T_COMMON_DEPT_map',nvl (DEPTID,'NULL'))",alias:"DEPTNAME_1",type:"string"),
(fname:"iilmap('T_XTSZ_BIND_map_1',DEPTID)",alias:"vf_DEPTID",type:"string"),
(fname:"iilmap('T_XTSZ_BIND_map_2',OWNERID)",alias:"vf_OWNERID",type:"string")
),
inputs: "T_GG_SJ_GOODS_dataset"
);
dataproc select T_GG_SJ_GOODS1_select
(
fields:
(
(fname:"DEPTID",type:"string"),
(fname:"DEPTNAME_1",type:"string"),
(fname:"GRADENUM",type:"string"),
(fname:"SHOPSIGNID",type:"string"),
(fname:"SHOPSIGNNAME",type:"string"),
(fname:"PRODAREAID",type:"string"),
(fname:"GOODSSTATENO",type:"string"),
(fname:"PRODAREANAME",type:"string"),
(fname:"FIRSTINDATE",type:"datetime"),
(fname:"KINDNO",type:"string"),
(fname:"KINDNAME",type:"string"),
(fname:"OWNERNAME",type:"string"),
(fname:"GOODSOWNERPACKNO",type:"string"),
(fname:"sum(NETWEIGHT)",alias:"SUMNETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"SUMGROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SUMSHEETNUM",type:"double"),
(fname:"vf_DEPTID",type:"string"),
(fname:"vf_OWNERID",type:"string")
),
inputs: "T_GG_SJ_GOODS_select",
conditions:"vf_DEPTID = '1'
AND vf_OWNERID = '1'
AND GOODSSTATENO = '10'
AND KINDNAME like '%热轧卷%'
AND SHOPSIGNNAME like '%SS330%'
AND GRADENUM like '%1037%'
AND PRODAREANAME like '%宝钢%'
AND GOODSOWNERPACKNO like '%15377%'
AND FIRSTINDATE >= TO_DATE ('2012-07-16', '%Y-&m-%d')
AND FIRSTINDATE < TO_DATE ('2016-07-16', '%Y-&m-%d') + 1",
group_by:(DEPTID,GRADENUM,SHOPSIGNID,SHOPSIGNNAME,PRODAREAID,PRODAREANAME,KINDNO,KINDNAME,OWNERNAME,GOODSOWNERPACKNO)
);
dataproc index T_GG_SJ_GOODS1_index
(
inputs: T_GG_SJ_GOODS1_select,
table: T_GG_SJ_GOODS1
);
dataproc doc T_GG_SJ_GOODS1_doc
(
inputs:T_GG_SJ_GOODS1_select,
table:T_GG_SJ_GOODS1,
format:T_GG_SJ_GOODS1_parser,
fields:("DEPTID","DEPTNAME_1","GRADENUM","SHOPSIGNID","SHOPSIGNNAME","PRODAREAID","PRODAREANAME","KINDNO","KINDNAME","OWNERNAME","GOODSOWNERPACKNO","SUMNETWEIGHT","SUMGROSSWEIGHT","SUMSHEETNUM","vf_DEPTID","vf_OWNERID")
);
end;
run job T_GG_SJ_GOODS_job (threads:8);
<file_sep>/complete/JINSHANG-110/del_3.sql
create job del_3_job(2)
begin
dataset file T_ZY_CK_OUTBILL_dataset_22
(
schema: T_ZY_CK_OUTBILL_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_ck_outbill/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc select union_select
(
inputs: "T_ZY_CK_OUTBILL_dataset_22",
fields:
(
(fname:"0",alias:"store_amount",type:"double"),
(fname:"0",alias:"store_in_amount",type:"double"),
(fname:"netweightsum",alias:"store_out_amount",type:"double"),
(fname:"0",alias:"store_process_amount",type:"double")
),
distinct: true,
data_opr:delete,
cache:true,
conditions:" deptid NOT LIKE '03%'
AND deptid NOT LIKE 'ZZ%'
AND deptid NOT LIKE 'MG%' "
);
dataproc statistics T_ZY_CK_OUTBILL_del_statistics
(
inputs:union_select,
table:union_all,
data_opr:delete
);
end;
run job del_3_job (threads:8);<file_sep>/uncomplete/JINSHANG-93/jinshang-93.sql
SELECT m.PROVIDER_NAME,
sum(td.weight_active/1000) WEIGHT_STRC,
min(t.time_receive),
CASE
WHEN min(t.time_receive)+7<sysdate THEN 1
ELSE 0
END flag
FROM wl.t_his_t_stacking_m t,
wl.t_his_t_stacking_d td,
wmsdba.t_si_logistics_ready_m m,
wmsdba.t_si_logistics_ready_d md
WHERE t.stacking_rec_num=td.stacking_rec_num
AND m.seg_no=md.seg_no
AND m.ready_num=md.ready_num
AND t.seg_no='00103'
AND m.seg_no='00103'
AND t.time_receive>=sysdate-30
AND m.time_receive>sysdate-30
AND t.optflag=0
AND t.order_num=m.order_num
AND t.seg_no=m.seg_no
AND td.pack_num=md.pack_num
GROUP BY m.PROVIDER_NAME;
<file_sep>/complete/JINSHANG-76/add.sql
create job t_common_dept_insert_q1(zykie)
begin
dataset file t_common_dept1_dataset
(
schema:T_COMMON_DEPT_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_common_dept/insert/insert.csv",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc syncher t_common_dept_syncher
(
inputs:t_common_dept1_dataset,
database:db,
operator:add,
table:T_COMMON_DEPT
);
end;
run job t_common_dept_insert_q1(threads:8);
create job t_bm_ui_configure_insert_q1(zykie)
begin
dataset file T_BM_UI_CONFIGURE1_dataset
(
schema:T_BM_UI_CONFIGURE_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_bm_ui_configure/insert/insert.csv",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc syncher t_common_dept_syncher
(
inputs:T_BM_UI_CONFIGURE1_dataset,
database:db,
operator:add,
table:T_BM_UI_CONFIGURE
);
end;
run job t_bm_ui_configure_insert_q1(threads:8);
create job t_gg_sj_goods_insert_q1(zykie)
begin
dataset file T_GG_SJ_GOODS1_dataset
(
schema:T_GG_SJ_GOODS_schema,
filename:"/home/natt/syncdata/oraclesyncdata/t_gg_sj_goods/insert/insert.csv",
serverid:0,
splitter:(block_size: 10000000)
);
dataproc select T_GG_SJ_GOODS1_select
(
fields:
(
(fname:"nvl (deptid,'NULL')",alias:"deptid",type:"str"),
(fname:"iilmap('T_COMMON_DEPT_map')",alias:"vf_deptname",type:"str"),
(fname:"nvl (gradenum, 'NULL')",alias:"gradenum",type:"str"),
(fname:"nvl (shopsignid, 'NULL')",alias:"shopsignid",type:"str"),
(fname:"nvl (shopsignname, 'NULL')",alias:"shopsignname",type:"str"),
(fname:"nvl (prodareaid, 'NULL')",alias:"prodareaid",type:"str"),
(fname:"nvl (prodareaname, 'NULL')",alias:"prodareaname",type:"str"),
(fname:"nvl (kindno, 'NULL')",alias:"kindno",type:"str"),
(fname:"nvl (kindname, 'NULL')",alias:"kindname",type:"str"),
(fname:"nvl (ownername, 'NULL')",alias:"ownername",type:"str"),
(fname:"nvl (goodsownerpackno, 'NULL')",alias:"goodsownerpackno",type:"str"),
(fname:"nvl (netweight, 0)",alias:"netweight",type:"str"),
(fname:"nvl (grossweight, 0)",alias:"grossweight",type:"str"),
(fname:"nvl (sheetnum, 0)",alias:"sheetnum",type:"str"),
(fname:"iilmap('T_BM_UI_CONFIGURE_map',1)",alias:"vf_count",type:"str")
),
inputs: "T_GG_SJ_GOODS1_dataset"
);
dataproc select T_GG_SJ_GOODS12_select
(
fields:
(
(fname:"deptid",type:"str"),
(fname:"vf_deptname",type:"str"),
(fname:"gradenum",type:"str"),
(fname:"shopsignid",type:"str"),
(fname:"shopsignname",type:"str"),
(fname:"prodareaid",type:"str"),
(fname:"prodareaname",type:"str"),
(fname:"kindno",type:"str"),
(fname:"kindname",type:"str"),
(fname:"ownername",type:"str"),
(fname:"goodsownerpackno",type:"str"),
(fname:"sum(netweight)",alias:"sumnetweight",type:"str"),
(fname:"sum(grossweight)",alias:"sumgrossweight",type:"str"),
(fname:"sum(sheetnum)",alias:"sumsheetnum",type:"str"),
(fname:"vf_count",type:"str"),
(fname:"case vf_count when '0' then '101%' else '101' end",alias:"vf_countcase",type:"str")
),
inputs: "T_GG_SJ_GOODS1_select",
group_by:(deptid,vf_deptname,gradenum,shopsignid,shopsignname,prodareaid,prodareaname,kindno,kindname,ownername,goodsownerpackno,vf_count,vf_countcase)
);
dataproc syncher T_GG_SJ_GOODS1_syncher
(
inputs: T_GG_SJ_GOODS12_select,
database:db,
operator:add,
table:T_GG_SJ_GOODS1
);
end;
run job t_gg_sj_goods_insert_q1(threads:8);<file_sep>/schema/T_COMMON_DEPT.sql
create schema T_COMMON_DEPT_schema
source type CSV
fields(
"deptid" type str,
"deptcd" type str,
"deptname" type str,
"deptlevel" type double,
"deptdesc" type str,
"deptorder" type double,
"parentdeptid" type str,
"level1deptid" type str,
"level1deptcd" type str,
"level1deptname" type str,
"level2deptid" type str,
"level2deptcd" type str,
"level2deptname" type str,
"level3deptid" type str,
"level3deptcd" type str,
"level3deptname" type str,
"level4deptid" type str,
"level4deptcd" type str,
"level4deptname" type str,
"r_record_create_date" type DATETIME,
"r_record_create_user" type str,
"r_record_update_date" type DATETIME,
"r_record_update_user" type str,
"r_record_is_deleted" type double,
"r_record_change_flag" type double,
"deptaddress" type str,
"depttype" type str default value '22',
"districtid" type str,
"districtname" type str,
"belongarea" type str,
"firstdis" type str,
"opselected" type str default value '0',
"opupload" type str,
"limittime" type DATETIME,
"contractno" type str,
"baosaas_customer_name" type str
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "NULL";
CREATE PARSER T_COMMON_DEPT_parser
TYPE rcd
SCHEMA T_COMMON_DEPT_schema;
CREATE TABLE T_COMMON_DEPT using T_COMMON_DEPT_parser;
CREATE INDEX T_COMMON_DEPT_index ON TABLE T_COMMON_DEPT (deptid);<file_sep>/uncomplete/JINSHANG-109/jinshang-109.sql
INSERT INTO SHOW.PDGM_KCAGE
SELECT deptid,
sum(a),
sum(b),
sum(c),
sum(d)
FROM
( SELECT deptid,
sum(netweight) a,
0 b,
0 c,
0 d
FROM wmsdba.t_gg_sj_goods t
WHERE goodsstateno=20
AND firstindate>=sysdate-30
AND exists
(SELECT 1
FROM WL.T_OWNER W
WHERE SEG_NO='00103'
AND T.DEPTID=W.DEPTID
AND t.ownerid=w.owner_id
AND deptid NOT LIKE 'A%'
AND deptid!='0301'
AND deptid!='4501')
GROUP BY deptid
UNION ALL SELECT deptid,
0 a,
sum(netweight) b,
0 c,
0 d
FROM wmsdba.t_gg_sj_goods t
WHERE goodsstateno=20
AND firstindate>=sysdate-60
AND firstindate<sysdate-30
AND exists
(SELECT 1
FROM WL.T_OWNER W
WHERE SEG_NO='00103'
AND T.DEPTID=W.DEPTID
AND t.ownerid=w.owner_id
AND deptid NOT LIKE 'A%'
AND deptid!='0301'
AND deptid!='4501')
GROUP BY deptid
UNION ALL SELECT deptid,
0 a,
0 b,
sum(netweight) c,
0 d
FROM wmsdba.t_gg_sj_goods t
WHERE goodsstateno=20
AND firstindate>=sysdate-180
AND firstindate<sysdate-60
AND exists
(SELECT 1
FROM WL.T_OWNER W
WHERE SEG_NO='00103'
AND T.DEPTID=W.DEPTID
AND t.ownerid=w.owner_id
AND deptid NOT LIKE 'A%'
AND deptid!='0301'
AND deptid!='4501')
GROUP BY deptid
UNION ALL SELECT deptid,
0 a,
0 b,
0 c,
sum(netweight) d
FROM wmsdba.t_gg_sj_goods t
WHERE goodsstateno=20
AND firstindate<sysdate-180
AND exists
(SELECT 1
FROM WL.T_OWNER W
WHERE SEG_NO='00103'
AND T.DEPTID=W.DEPTID
AND t.ownerid=w.owner_id
AND deptid NOT LIKE 'A%'
AND deptid!='0301'
AND deptid!='4501')
GROUP BY deptid)
GROUP BY deptid;
<file_sep>/uncomplete/JINSHANG-83/jinshang-83.sql
SELECT sum(a)
FROM
(SELECT sum(netweightsum) a
FROM t_zy_rk_inbill
WHERE r_record_create_date>=to_date(to_char(sysdate,'yyyy')||'-1-1','yyyy-mm-dd')
UNION SELECT sum(netweightsum) a
FROM t_zy_ck_outbill
WHERE r_record_create_date>=to_date(to_char(sysdate,'yyyy')||'-1-1','yyyy-mm-dd'));
<file_sep>/uncomplete/JINSHANG-80/jinshang-80.sql
UPDATE SHOW.SEGWEIGHT
SET stackweight=0,
tidanweight=0,
readyweight=0
WHERE 1=1;
UPDATE SHOW.SEGWEIGHT s
SET STACKWEIGHT=
(SELECT STACKWEIGHT
FROM
( SELECT extsysstackid seg_no,
SUM(STACKING_WEIGHT) STACKWEIGHT
FROM t_gg_tx_dl_in_sm a
WHERE a.insert_time>=to_date(to_char(sysdate,'yyyy-mm-dd'),'yyyy-mm-dd')
AND exists
(SELECT 1
FROM SHOW.SEGWEIGHT b
WHERE a.extsysstackid=b.seg_no)
GROUP BY extsysstackid) t
WHERE s.seg_no=t.seg_no);
UPDATE SHOW.SEGWEIGHT s
SET TIDANWEIGHT=
(SELECT STACKWEIGHT
FROM
( SELECT seg_no,
SUM(UNIT_WEIGHT) STACKWEIGHT
FROM t_gg_tx_dl_bil_d a
WHERE a.insert_time>=to_date(to_char(sysdate,'yyyy-mm-dd'),'yyyy-mm-dd')
AND exists
(SELECT 1
FROM SHOW.SEGWEIGHT b
WHERE a.seg_no=b.seg_no)
GROUP BY seg_no) t
WHERE s.seg_no=t.seg_no);
UPDATE SHOW.SEGWEIGHT s
SET READYWEIGHT=
(SELECT STACKWEIGHT
FROM
( SELECT seg_no,
SUM(WEIGHT_READY_TOT) STACKWEIGHT
FROM t_si_logistics_ready_m a
WHERE a.TIME_RECEIVE>=to_date(to_char(sysdate,'yyyy-mm-dd'),'yyyy-mm-dd')
AND exists
(SELECT 1
FROM SHOW.SEGWEIGHT b
WHERE a.seg_no=b.seg_no)
GROUP BY seg_no) t
WHERE s.seg_no=t.seg_no);
<file_sep>/schema/T_CHART_DETAILSTORAGE.sql
create schema T_CHART_DETAILSTORAGE_schema
source type CSV
fields(
"owner_num" type str,
"deptid" type str,
"deptname" type str,
"sumdate" type str,
"sumcount" type double,
"sumweight" type double
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "NULL";
CREATE PARSER T_CHART_DETAILSTORAGE_parser
TYPE rcd
SCHEMA T_CHART_DETAILSTORAGE_schema;
CREATE TABLE T_CHART_DETAILSTORAGE using T_CHART_DETAILSTORAGE_parser;
CREATE INDEX T_CHART_DETAILSTORAGE_index ON TABLE T_CHART_DETAILSTORAGE (owner_num);<file_sep>/complete/JINSHANG-110/del_2.sql
create job del_2_job(2)
begin
dataset file T_ZY_RK_INBILL_dataset_22
(
schema: T_ZY_RK_INBILL_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_rk_inbill/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc select union_select
(
inputs: "T_ZY_RK_INBILL_dataset_22",
fields:
(
(fname:"0",alias:"store_amount",type:"double"),
(fname:"netweightsum",alias:"store_in_amount",type:"double"),
(fname:"0",alias:"store_out_amount",type:"double"),
(fname:"0",alias:"store_process_amount",type:"double")
),
distinct: true,
data_opr:delete,
cache:true,
conditions:" deptid NOT LIKE '03%'
AND deptid NOT LIKE 'ZZ%'
AND deptid NOT LIKE 'MG%' "
);
dataproc statistics T_ZY_RK_INBILL_del_statistics
(
inputs:union_select,
table:union_all,
data_opr:delete
);
end;
run job del_2_job (threads:8);<file_sep>/complete/JINSHANG-75/1_schema.sql
create database db;
use db;
create schema t_common_dept_schema
source type csv
FIELDS(
"DEPTID" TYPE string,
"DEPTCD" TYPE string,
"DEPTNAME" TYPE string,
"DEPTLEVEL" TYPE double,
"DEPTDESC" TYPE string,
"DEPTORDER" TYPE double,
"PARENTDEPTID" TYPE string,
"LEVEL1DEPTID" TYPE string,
"LEVEL1DEPTCD" TYPE string,
"LEVEL1DEPTNAME" TYPE string,
"LEVEL2DEPTID" TYPE string,
"LEVEL2DEPTCD" TYPE string,
"LEVEL2DEPTNAME" TYPE string,
"LEVEL3DEPTID" TYPE string,
"LEVEL3DEPTCD" TYPE string,
"LEVEL3DEPTNAME" TYPE string,
"LEVEL4DEPTID" TYPE string,
"LEVEL4DEPTCD" TYPE string,
"LEVEL4DEPTNAME" TYPE string,
"R_RECORD_CREATE_DATE" TYPE datetime,
"R_RECORD_CREATE_USER" TYPE string,
"R_RECORD_UPDATE_DATE" TYPE datetime,
"R_RECORD_UPDATE_USER" TYPE string,
"R_RECORD_IS_DELETED" TYPE double,
"R_RECORD_CHANGE_FLAG" TYPE double,
"DEPTADDRESS" TYPE string,
"DEPTTYPE" TYPE string default value '22',
"DISTRINGICTID" TYPE string,
"DISTRINGICTNAME" TYPE string,
"BELONGAREA" TYPE string,
"FIRSTDIS" TYPE string,
"OPSELECTED" TYPE string default value '0',
"OPUPLOAD" TYPE string,
"LIMITTIME" TYPE datetime,
"CONTRACTNO" TYPE string,
"BAOSAAS_CUSTOMER_NAME" TYPE string
)
RECORD DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
create parser t_common_dept_parser
type rcd
schema t_common_dept_schema;
create table t_common_dept using t_common_dept_parser;
create index t_common_dept_index on table t_common_dept PRIMARY KEY(DEPTID);
create map t_common_dept_map on table t_common_dept
key (DEPTID)
value (DEPTNAME)
type string;
create schema t_gg_sj_goods_schema
source type csv
FIELDS(
"GOODSID" TYPE string,
"DEPTID" TYPE string,
"PACKNUM" TYPE string,
"BARCODE" TYPE string,
"GOODSLOCID" TYPE string,
"STOCKTYPE" type string,
"OWNERID" TYPE string,
"OWNERNAME" TYPE string,
"GOODSOWNERMANAGENO" TYPE string,
"INBILLID" TYPE string,
"GOODSOWNERPACKNO" TYPE string,
"MANAGENO" TYPE string,
"FEEBEGINDATE" TYPE datetime,
"CONTRACTID" TYPE string,
"GOODSSTATENO" TYPE string,
"GOODSSTATENAME" TYPE string,
"CHANGEOWNERTIMES" TYPE double default value '0',
"GOODSTYPE" TYPE string,
"GOODSTYPENAME" TYPE string,
"KINDNO" TYPE string,
"KINDNAME" TYPE string,
"SHOPSIGNID" TYPE string,
"SHOPSIGNNAME" TYPE string,
"PRODAREAID" TYPE string,
"PRODAREANAME" TYPE string,
"GOODSBATCHNO" TYPE string,
"PRODSTOVENO" TYPE string,
"GRADENUM" TYPE string,
"GRADENUMTHICK" TYPE double,
"GRADENUMWIDTH" TYPE double,
"GRADENUMLENGTH" TYPE double,
"GROSSWEIGHT" TYPE double,
"NETWEIGHT" TYPE double,
"POUNDWEIGHT" TYPE double,
"CHECKWEIGHT" TYPE double,
"SHEETNUM" TYPE double default value '1',
"UNIT" TYPE string,
"PACKTYPE" TYPE string,
"STOCKAREAID" TYPE string,
"QUALITYMEMO" TYPE string,
"FIRSTINOWNERID" TYPE string,
"FIRSTINDATE" TYPE datetime,
"OUTBILLID" TYPE string,
"LDGLISTID" TYPE string,
"MATERIALGOODSID" TYPE string,
"INTYPE" type string,
"OUTTYPE" TYPE string,
"BILLSTATE" TYPE double,
"OUTBILLTIME" TYPE datetime,
"MEMO" TYPE string,
"EXTSYSPLANOBJECTID" TYPE string,
"EXTSYSKINDID" TYPE string,
"EXTSYSSHOPSIGNID" TYPE string,
"EXTSYSPRODAREAID" TYPE string,
"FOREIGNLISTNO" TYPE string,
"SENDFLAG" TYPE double,
"R_RECORD_CREATE_DATE" TYPE datetime,
"R_RECORD_CREATE_USER" TYPE string,
"R_RECORD_UPDATE_DATE" TYPE datetime,
"R_RECORD_UPDATE_USER" TYPE string,
"R_RECORD_IS_DELETED" TYPE double,
"INCUSTTIME" TYPE datetime,
"SIDEMARK" TYPE string,
"TEMP" TYPE string,
"LENGTH_PRODUCT_MAX" TYPE double,
"HEAT_NUM" TYPE string,
"TEST_LOT_NUM" TYPE string,
"R_RECORD_CHANGE_FLAG" TYPE double,
"SDPRICE" TYPE string,
"EXTSYSGRADENUM" TYPE string,
"COLLECTIONMANNAME" TYPE string,
"LOCKFLAG" TYPE string,
"CHECK_TYPE" TYPE string,
"OUTDATE" TYPE datetime,
"YFWEIGHT" TYPE double,
"YFSHEET" TYPE double,
"PREGOODSID" TYPE string,
"ISSPLIT" TYPE double,
"GRADENUMXH" TYPE double,
"GRADENUMWHDW" TYPE string,
"SPLITTIME" TYPE double,
"ISCLEAR" TYPE double,
"SINGLESHEETNUM" TYPE double,
"GRADENUMHZ" TYPE string,
"GRADENUMTHICKHZ" TYPE double,
"SINGLESHEET" TYPE double,
"LOCLV1" TYPE string,
"SINGLESHEETUNIT" TYPE string,
"DCEMP" TYPE string,
"QZEMP" TYPE string,
"DCEMP2" TYPE string,
"SHIPNO" TYPE string,
"SHIPNAME" TYPE string,
"SHIPCD" TYPE string,
"SHIPLEVEL" TYPE string,
"CMMEMO" TYPE string,
"CMMEMOCK" TYPE string,
"SHOPSIGNCD" TYPE string,
"PROCESSTYPENAME" TYPE string,
"PACKTYPENAME" TYPE string,
"SORT_EMP" TYPE string,
"CUSTOMERID" TYPE string,
"LVGOODSID" TYPE string,
"DISPERSESHEET" TYPE double,
"DBDEPTID" TYPE string,
"DBCUSTOMER" TYPE string,
"DBGULENDER" TYPE string,
"LOCKED_OBJECT" TYPE string,
"LOCKED_DATE" TYPE datetime,
"UNLOCKED_DATE" TYPE datetime,
"PREOWNERNAME" TYPE string,
"PLY" TYPE double default value '0',
"TDSTATE" type string default value '1',
"OPSELECTED" TYPE string,
"OPLOCK" TYPE string,
"OPINUPLOAD" TYPE string,
"OPCHANGEUPLOAD" TYPE string,
"OPINBATCHID" TYPE string,
"OPCHANGEBATCHID" TYPE string,
"OPINUPLOAD_DATE" TYPE datetime,
"OUT_PACKAGE_STATUS" TYPE string,
"OUT_PACKAGE_DESC" TYPE string,
"GMPACKTYPE" TYPE string,
"HANDNO" TYPE string,
"SAVENO" TYPE string,
"DRAWNO" TYPE string,
"DANGEROUSLEVEL" TYPE string,
"ACCEPTANCECRITERIA" TYPE string,
"SHIPPINGMARK" TYPE string,
"LOCLV2" TYPE string,
"PAYEDFLAG" TYPE string,
"ORDERGOODSOWNERID" TYPE string,
"ORDERGOODSOWNERNAME" TYPE string,
"VF_DEPTNAME" type `expr` data type string value iilmap("t_common_dept_map",DEPTID)
)
RECORD DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
create parser t_gg_sj_goods_parser
type rcd
schema t_gg_sj_goods_schema;
create table t_gg_sj_goods using t_gg_sj_goods_parser;
create index t_gg_sj_goods_index on table t_gg_sj_goods PRIMARY KEY(GOODSID);
<file_sep>/uncomplete/JINSHANG-98/jinshang-98.sql
SELECT t.DEPTID,
t.PLANENDDATE,
t.OUTCARBILLTIME,
t.PROCESSTYPENAME,
t.PROCESSID,
t.PROCESSDEPT,
t.PROCESSDEPTNAME,
t.PROCESSCUSTOMNO,
t.PROCESSCUSTOMNAME,
t.PROCESSMOLDNAME,
t.SETTLEDEPTNO,
t.SETTLEDEPTNAME,
t.M_MANAGENO,
t.M_GRADENUM,
t.M_NETWEIGHT,
t.NETWEIGHT,
t.A_GRADENUM,
t.A_GRADENUM2,
t.A_SHOPSIGNNAME,
t.s_COUNTUNIT,
t.s_SETTLENUM,
t.s_SETTLESUM,
t.s_INVOICEKINDID,
t.s_INVOICEKINDNAME,
t.FACTSUM,
t.LASTSUM,
t.PACKTYPENAME,
t.settle_date,
t.customerid,
t.FEETYPE,
t.FEETYPENAME,
t.JSMEMO ,
t.PRODAREANAME,
t.chanxian,
t.Goodslocid
FROM
(SELECT a.DEPTID,
a.PROCESSID,
a.PROCESSDEPT,
a.PROCESSDEPTNAME,
a.PROCESSCUSTOMNO,
a.PROCESSCUSTOMNAME,
a.SETTLEDEPTNO,
a.SETTLEDEPTNAME,
a.PROCESSTYPENAME,
a.PROCESSMOLDNAME,
a.PLANENDDATE,
a.OUTCARBILLTIME,
a.PREWEIGHTSUM,
a.M_MANAGENO,
a.M_GRADENUM,
a.PACKNUM_NUM,
a.NETWEIGHT,
a.M_NETWEIGHT,
a.A_GRADENUM,
a.A_GRADENUM2,
a.A_SHOPSIGNNAME,
b.s_SETTLENUM,
b.s_SETTLESUM,
b.s_COUNTUNIT,
b.s_INVOICEKINDID,
b.s_INVOICEKINDNAME,
b.factsum,
b.s_SETTLESUM-b.factsum Lastsum,
a.packtypename,
a.CUSTOMERID,
b.CREATDATE settle_date,
b.FEETYPE,
b.FEETYPENAME,
b.JSMEMO,
a.PRODAREANAME,
a.chanxian,
a.Goodslocid
FROM
(SELECT P.DEPTID,
P.PROCESSID,
P.PROCESSDEPT,
P.PROCESSDEPTNAME,
P.PROCESSCUSTOMNO,
P.PROCESSCUSTOMNAME,
P.SETTLEDEPTNO,
P.SETTLEDEPTNAME,
P.PROCESSTYPENAME,
P.PROCESSMOLDNAME,
P.PLANENDDATE,
p.preweightsum,
p.packtypename,
p.customerid,
(SELECT MAX(JM.MANAGENO)
FROM wmsdba.T_ZY_JG_PROCMATERIALDTL JM
WHERE JM.DEPTID = P.DEPTID
AND JM.PROCESSID = P.PROCESSID) AS M_MANAGENO,
--原料采购资源号
(SELECT MAX(JM.GRADENUM) AS GRADENUM
FROM wmsdba.T_ZY_JG_PROCMATERIALDTL JM
WHERE JM.DEPTID = P.DEPTID
AND JM.PROCESSID = P.PROCESSID) AS M_GRADENUM,
--原料规格
COUNT(JA.PACKNUM) AS PACKNUM_NUM,
--成品数量
sum(JA.NETWEIGHT) AS NETWEIGHT,
--成品重量
(SELECT SUM(JM.NETWEIGHT) AS NETWEIGHT
FROM wmsdba.T_ZY_JG_PROCMATERIALDTL JM
WHERE JM.DEPTID = P.DEPTID
AND JM.PROCESSID = P.PROCESSID) AS M_NETWEIGHT,
--原料重量
(SELECT JM.GRADENUM
FROM wmsdba.T_ZY_JG_PROCARTICLEDTL JM
WHERE JM.DEPTID = P.DEPTID
AND JM.PROCESSID = P.PROCESSID
AND rownum=1) AS A_GRADENUM,
(SELECT JM.GRADENUM
FROM wmsdba.T_ZY_JG_PROCARTICLEDTL JM
WHERE JM.DEPTID = P.DEPTID
AND JM.PROCESSID = P.PROCESSID
AND rownum=1
AND jm.goodstypename='加工成品') AS A_GRADENUM2,
min(JA.SHOPSIGNNAME) AS A_SHOPSIGNNAME,
--成品牌号
max(JA.FIRSTINDATE) AS OUTCARBILLTIME,
--成品验收日期
max(JA.PRODAREANAME) AS PRODAREANAME,
--产地
max(P.Chanxian) AS Chanxian,
--产线
max(JA.Goodslocid) AS Goodslocid--库位
FROM wmsdba.T_ZY_JG_PROCESS P,
wmsdba.T_ZY_JG_PROCARTICLEDTL JA
WHERE P.DEPTID = JA.DEPTID
AND P.PROCESSID = JA.PROCESSID
AND P.Processstate=1
AND JA.GOODSTYPENAME!='加工余料'
GROUP BY P.DEPTID,
P.PROCESSID,
P.PROCESSDEPT,
P.PROCESSDEPTNAME,
P.PROCESSCUSTOMNO,
P.PROCESSCUSTOMNAME,
P.SETTLEDEPTNO,
P.SETTLEDEPTNAME,
P.PROCESSTYPENAME,
P.PROCESSMOLDNAME,
P.PLANENDDATE,
p.preweightsum,
p.customerid,
p.packtypename) A,
(SELECT T.DEPTID,
T.BUSINESSNO,
SUM(T.SETTLENUM) AS s_SETTLENUM,
SUM(T.SETTLESUM) AS s_SETTLESUM,
MAX(T.COUNTUNIT) AS s_COUNTUNIT,
T.INVOICEKINDID AS s_INVOICEKINDID,
T.INVOICEKINDNAME AS s_INVOICEKINDNAME,
mst.Factsum,
mst.CREATDATE,
t.FEETYPE,
t.FEETYPENAME,
MAX(T.MEMO) JSMEMO
FROM WMSDBA.T_ZY_JS_SETTLEMENT MST,
WMSDBA.T_ZY_JS_SETTLEMENTDETAIL T
WHERE T.DEPTID=MST.Deptid
AND T.Settlecd=MST.Settlecd
GROUP BY T.DEPTID,
T.BUSINESSNO,
T.INVOICEKINDID,
T.INVOICEKINDNAME,
mst.Factsum,
T.Settlecd,
mst.CREATDATE,
t.feetype,
t.feetypename) B
WHERE A.DEPTID = B.DEPTID
AND A.PROCESSID = B.BUSINESSNO) t
WHERE t.DEPTID=:dp
AND t.processdept='KH03632'
AND settle_date>=to_date('2015/7/3','yyyy-mm-dd')
AND settle_date<to_date('2015/8/4','yyyy-mm-dd')
AND OUTCARBILLTIME>=to_date('2015/7/3','yyyy-mm-dd')
AND OUTCARBILLTIME<to_date('2015/8/4','yyyy-mm-dd')
AND FeeType = '001002'
ORDER BY t.PLANENDDATE ;
<file_sep>/schema/T_ZY_JG_PROCESS.sql
create schema T_ZY_JG_PROCESS_schema
SOURCE TYPE CSV
FIELDS(
"processid" type str,
"deptid" type str,
"customerid" type str,
"processdept" type str,
"processdeptname" type str,
"processtype" type str,
"processtypename" type str,
"processmold" type str,
"processmoldname" type str,
"processstate" type double,
"planenddate" type datetime,
"kindno" type str,
"kindname" type str,
"packtype" type str,
"packtypename" type str,
"woodtype" type str,
"woodtypename" type str,
"straptype" type str,
"straptypename" type str,
"processprecision" type str,
"orderinfo" type str,
"fee" type double,
"length" type double,
"weigtht" type double,
"sheet" type double,
"piecenum" type double,
"outcarbilltime" type datetime,
"outcarbillempno" type str,
"outcarbillempname" type str,
"planno" type str,
"preweightsum" type double,
"meno" type str,
"gradenumrange" type str,
"gradenumrangename" type str,
"thickrange" type str,
"thickrangename" type str,
"r_record_create_date" type datetime,
"r_record_create_user" type str,
"r_record_update_date" type datetime,
"r_record_update_user" type str,
"r_record_is_deleted" type double,
"r_record_change_flag" type double,
"density" type double,
"processcustomno" type str,
"processcustomname" type str,
"settledeptno" type str,
"settledeptname" type str,
"settle_staus" type str,
"settle_staus_name" type str,
"settle_no" type str,
"settle_date" type datetime,
"acceptancer" type str,
"acceptance_time" type datetime,
"chanxian" type str,
"mddeptname" type str,
"articleys" type str,
"materialgradenum" type str,
"materialshopsign" type str,
"cbxjgtype" type str,
"jgmessage" type str,
"jgstatus" type str,
"articlefee" type str,
"issettled" type double,
"specialdown" type str,
"articlesheet" type double,
"articleweight" type double,
"username" type str,
"jfsendflag" type double,
"isreceived" type str,
"specialmemo" type str,
"toprocessid" type str,
"jxlocked" type str
)
SCHEMA DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_ZY_JG_PROCESS_parser
TYPE rcd
SCHEMA T_ZY_JG_PROCESS_schema;
CREATE TABLE T_ZY_JG_PROCESS using T_ZY_JG_PROCESS_parser;
create index T_ZY_JG_PROCESS_index on table T_ZY_JG_PROCESS(processid);<file_sep>/uncomplete/JINSHANG-95/jinshang-95.sql
JINSHANG-95
SELECT rptdate,
Sum(nt) nt,
Sum(injs) injs,
Sum(OUTWEIGHT) OUTWEIGHT,
Sum(outjs) outjs
FROM (SELECT To_char(FirstInDate, 'yyyy-mm-dd') AS rptdate,
Sum(netweight) AS nt,
Count(*) AS injs,
0 OUTWEIGHT,
0 outjs
FROM T_ZY_RK_InBillDetail
WHERE ( goodsstateno = '20' )
AND ( DeptID = '0301' )
AND ( FirstInDate >= To_date('2015/7/1', 'yyyy-mm-dd') )
AND ( FirstInDate < To_date('2015/9/1', 'yyyy-mm-dd') )
AND ownerid = 'KH00001'
AND ( 1 = 2
OR MANAGENO LIKE 'M%')
GROUP BY To_char(FirstInDate, 'yyyy-mm-dd')
UNION ALL
SELECT To_char(b.changedate, 'yyyy-mm-dd') AS rptdate,
Sum(netweight) AS nt,
Count(*) AS injs,
0 OUTWEIGHT,
0 outjs
FROM T_ZY_GH_ChangeOwnerDetail a,
T_ZY_GH_ChangeOwnerlist b
WHERE a.deptid = b.deptid
AND a.changeownerno = b.changeownerid
AND a.DeptID = '0301'
AND newownerno = 'KH00001'
AND ( 1 = 2
OR a.premanagenum LIKE 'M%' )
AND changedate >= To_date('2015/7/1', 'yyyy-mm-dd')
AND changedate < To_date('2015/9/1', 'yyyy-mm-dd')
GROUP BY To_char(b.changedate, 'yyyy-mm-dd')
UNION ALL
SELECT To_char(bill_date, 'yyyy-mm-dd') AS rptdate,
0 nt,
0 injs,
Sum(unit_weight) AS OUTWEIGHT,
Count(*) AS outjs
FROM (SELECT a.bill_num,
a.bill_date,
pack_id pack_num,
plan_weight unit_weight
FROM (SELECT *
FROM T_GG_TX_DL_BIL_M
WHERE ( DeptID = '0301' )
AND ( OWNER_NUM = 'GM0' )
AND ( BILL_DATE >= To_date('2015/7/1', 'yyyy-mm-dd') )
AND ( BILL_DATE < To_date('2015/9/1', 'yyyy-mm-dd') )
AND billstate >= '20'
AND ( 1 = 2
OR resource_num LIKE 'M%' )) a
LEFT JOIN wl.t_s_bill_d b
ON a.seg_no = b.seg_no
AND a.resource_num = b.factory_product_id
AND a.BILL_NUM = b.BILL_ID) t3
GROUP BY To_char(bill_date, 'yyyy-mm-dd'))
GROUP BY rptdate;
1.里层条件不能永远都写死,必须传到外层给我们JQL输入的where条件做过滤。
<file_sep>/complete/JINSHANG-110/add_3.sql
create job add_3_job(2)
begin
dataset file T_ZY_CK_OUTBILL_dataset_11
(
schema: T_ZY_CK_OUTBILL_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_ck_outbill/insert/insert.csv",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc select union_select
(
inputs: "T_ZY_CK_OUTBILL_dataset_11",
fields:
(
(fname:"0",alias:"store_amount",type:"double"),
(fname:"0",alias:"store_in_amount",type:"double"),
(fname:"netweightsum",alias:"store_out_amount",type:"double"),
(fname:"0",alias:"store_process_amount",type:"double")
),
distinct: true,
cache:true,
conditions:" deptid NOT LIKE '03%'
AND deptid NOT LIKE 'ZZ%'
AND deptid NOT LIKE 'MG%' "
);
dataproc statistics T_ZY_CK_OUTBILL_add_statistics
(
inputs:union_select,
table:union_all
);
end;
run job add_3_job (threads:8);<file_sep>/uncomplete/JINSHANG-93/T_HIS_T_STACKING_D.sql
CREATE SCHEMA T_HIS_T_STACKING_D_schema
SOURCE TYPE CSV
FIELDS(
"manu_id" type str,
"stacking_rec_num" type str,
"pick_num" type str,
"pack_num" type str,
"weight_active" type double,
"weight_theo" type double,
"n_pack" type double,
"time_receive" type datetime,
"time_proc" type datetime,
"proc_mark" type str,
"error_text1" type str,
"error_text2" type str,
"ws_flag" type str default value '0',
"ws_datat" type datetime,
"seg_no" type str,
"downloadflag" type str default value '0',
"length_product" type double,
"length_product_max" type double,
"length_product_min" type double,
"optflag" type str,
"heat_num" type str,
"test_lot_num" type str,
"order_num" type str,
"spec1" type str,
"spec3" type str,
"spec5" type str,
"ppflag" type str default value '0',
"scan_flag" type str default value '0'
)
SCHEMA DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_HIS_T_STACKING_D_parser
TYPE rcd
SCHEMA T_HIS_T_STACKING_D_schema;
CREATE TABLE T_HIS_T_STACKING_D using T_HIS_T_STACKING_D_parser;
CREATE INDEX T_HIS_T_STACKING_D_index ON TABLE T_HIS_T_STACKING_D (manu_id); <file_sep>/complete/JINSHANG-102/job7.sql
create job 7_job(8)
begin
dataset file T_ZY_JG_PROCMATERIALDTL_dataset
(
schema: T_ZY_JG_PROCMATERIALDTL_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_JG_PROCMATERIALDTL.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_JG_PROCMATERIALDTL_syncher
(
inputs: T_ZY_JG_PROCMATERIALDTL_dataset,
database:db,
operation:add,
table:T_ZY_JG_PROCMATERIALDTL,
stream:true,
services :
(
(dataprocname : T_ZY_JG_PROCMATERIALDTL_dataset,
serviceid: idx_job)
)
);
end;
run job 7_job (threads:8);
<file_sep>/complete/JINSHANG-82/jinshang-82.sql
SELECT count(DISTINCT deptid)
FROM t_zy_jg_process;
SELECT count(DISTINCT t.destination_mobile)
FROM xt.t_ss_sms_send_log t;
SELECT count(DISTINCT t.kindname)
FROM t_gg_sj_producttype t;
SELECT count(DISTINCT t.ownername)
FROM t_gg_sj_customer t;
<file_sep>/uncomplete/JINSHANG-103/jinshang-103.sql
SELECT sum(netweight),
sum(sheetnum),
sum(kdwt),
sum(zrrk),
sum(jrrk),
sum(zrck),
sum(jrck),
sum(byrk),
sum(byck),
sum(warn)
FROM
( SELECT sum(netweight) netweight,
sum(sheetnum) sheetnum,
0 kdwt,
0 zrrk,
0 jrrk,
0 zrck,
0 jrck,
0 byrk,
0 byck,
0 warn
FROM wmsdba.t_gg_sj_goods t
WHERE goodsstateno=20
AND deptid='0106'
AND exists
(SELECT 1
FROM WL.T_OWNER W
WHERE SEG_NO='00103'
AND T.DEPTID=W.DEPTID
AND t.ownerid=w.owner_id)
UNION ALL SELECT 0,
0,
sum(netweight),
0,
0,
0,
0,
0,
0,
0 warn
FROM wmsdba.t_gg_sj_goods t
WHERE goodsstateno=20
AND deptid='0106'
AND exists
(SELECT 1
FROM WL.T_OWNER W
WHERE SEG_NO='00103'
AND T.DEPTID=W.DEPTID
AND t.ownerid=w.owner_id)
AND exists
(SELECT 1
FROM wl.t_s_bill_d d
WHERE t.packnum=d.pack_id
AND d.seg_no='00103')
UNION ALL SELECT 0,
0,
0,
sum(netweightsum),
0,
0,
0,
0,
0,
0
FROM wmsdba.t_zy_rk_inbill t
WHERE deptid='0106'
AND r_record_create_date>=to_date(to_char(sysdate-1,'yyyy-mm-dd'),'yyyy-mm-dd')
AND r_record_create_date<to_date (to_char(sysdate,'yyyy-mm-dd'),'yyyy-mm-dd')
AND EXISTS
(SELECT 1
FROM WL.T_OWNER W
WHERE SEG_NO='00103'
AND T.DEPTID=W.DEPTID
AND t.inbillownerno=w.owner_id)
UNION ALL SELECT 0,
0,
0,
0,
sum(netweightsum),
0,
0,
0,
0,
0
FROM wmsdba.t_zy_rk_inbill t
WHERE deptid='0106'
AND r_record_create_date>=to_date(to_char(sysdate,'yyyy-mm-dd'),'yyyy-mm-dd')
AND EXISTS
(SELECT 1
FROM WL.T_OWNER W
WHERE SEG_NO='00103'
AND T.DEPTID=W.DEPTID
AND t.inbillownerno=w.owner_id)
UNION ALL SELECT 0,
0,
0,
0,
0,
sum(netweightsum),
0,
0,
0,
0
FROM wmsdba.t_zy_ck_outbill t
WHERE deptid='0106'
AND r_record_create_date>=to_date(to_char(sysdate-1,'yyyy-mm-dd'),'yyyy-mm-dd')
AND r_record_create_date<to_date (to_char(sysdate,'yyyy-mm-dd'),'yyyy-mm-dd')
AND EXISTS
(SELECT 1
FROM WL.T_OWNER W
WHERE SEG_NO='00103'
AND T.DEPTID=W.DEPTID
AND t.OUTBILLOWNERNO=w.owner_id)
UNION ALL SELECT 0,
0,
0,
0,
0,
0,
sum(netweightsum),
0,
0,
0
FROM wmsdba.t_zy_ck_outbill t
WHERE deptid='0106'
AND r_record_create_date>=to_date(to_char(sysdate,'yyyy-mm-dd'),'yyyy-mm-dd')
AND EXISTS
(SELECT 1
FROM WL.T_OWNER W
WHERE SEG_NO='00103'
AND T.DEPTID=W.DEPTID
AND t.OUTBILLOWNERNO=w.owner_id)
UNION ALL SELECT 0,
0,
0,
0,
0,
0,
0,
sum(netweightsum),
0,
0
FROM wmsdba.t_zy_rk_inbill t
WHERE deptid='0106'
AND r_record_create_date>=to_date(to_char(sysdate,'yyyy-mm'),'yyyy-mm')
AND EXISTS
(SELECT 1
FROM WL.T_OWNER W
WHERE SEG_NO='00103'
AND T.DEPTID=W.DEPTID
AND t.inbillownerno=w.owner_id)
UNION ALL SELECT 0,
0,
0,
0,
0,
0,
0,
0,
sum(netweightsum),
0
FROM wmsdba.t_zy_ck_outbill t
WHERE deptid='0106'
AND r_record_create_date>=to_date(to_char(sysdate,'yyyy-mm'),'yyyy-mm')
AND EXISTS
(SELECT 1
FROM WL.T_OWNER W
WHERE SEG_NO='00103'
AND T.DEPTID=W.DEPTID
AND t.OUTBILLOWNERNO=w.owner_id)
UNION ALL SELECT 0,
0,
0,
0,
0,
0,
0,
0,
0,
storelimit
FROM wmsdba.t_wl_store t
WHERE DEPT_ID='0106');
<file_sep>/schema/T_GG_SJ_GOODS.sql
create schema T_GG_SJ_GOODS_schema
source type CSV
fields(
"goodsid" type str,
"deptid" type str,
"packnum" type str,
"barcode" type str,
"goodslocid" type str,
"stocktype" type str,
"ownerid" type str,
"ownername" type str,
"goodsownermanageno" type str,
"inbillid" type str,
"goodsownerpackno" type str,
"manageno" type str,
"feebegindate" type DATETIME,
"contractid" type str,
"goodsstateno" type str,
"goodsstatename" type str,
"changeownertimes" type double default value '0',
"goodstype" type str,
"goodstypename" type str,
"kindno" type str,
"kindname" type str,
"shopsignid" type str,
"shopsignname" type str,
"prodareaid" type str,
"prodareaname" type str,
"goodsbatchno" type str,
"prodstoveno" type str,
"gradenum" type str,
"gradenumthick" type double,
"gradenumwidth" type double,
"gradenumlength" type double,
"grossweight" type double,
"netweight" type double,
"poundweight" type double,
"checkweight" type double,
"sheetnum" type double default value '1',
"unit" type str,
"packtype" type str,
"stockareaid" type str,
"qualitymemo" type str,
"firstinownerid" type str,
"firstindate" type DATETIME,
"outbillid" type str,
"ldglistid" type str,
"materialgoodsid" type str,
"intype" type str,
"outtype" type str,
"billstate" type double,
"outbilltime" type DATETIME,
"memo" type str,
"extsysplanobjectid" type str,
"extsyskindid" type str,
"extsysshopsignid" type str,
"extsysprodareaid" type str,
"foreignlistno" type str,
"sendflag" type double,
"r_record_create_date" type DATETIME,
"r_record_create_user" type str,
"r_record_update_date" type DATETIME,
"r_record_update_user" type str,
"r_record_is_deleted" type double,
"incusttime" type DATETIME,
"sidemark" type str,
"temp" type str,
"length_product_max" type double,
"heat_num" type str,
"test_lot_num" type str,
"r_record_change_flag" type double,
"sdprice" type str,
"extsysgradenum" type str,
"collectionmanname" type str,
"lockflag" type str,
"check_type" type str,
"outdate" type DATETIME,
"yfweight" type double,
"yfsheet" type double,
"pregoodsid" type str,
"issplit" type double,
"gradenumxh" type double,
"gradenumwhdw" type str,
"splittime" type double,
"isclear" type double,
"singlesheetnum" type double,
"gradenumhz" type str,
"gradenumthickhz" type double,
"singlesheet" type double,
"loclv1" type str,
"singlesheetunit" type str,
"dcemp" type str,
"qzemp" type str,
"dcemp2" type str,
"shipno" type str,
"shipname" type str,
"shipcd" type str,
"shiplevel" type str,
"cmmemo" type str,
"cmmemock" type str,
"shopsigncd" type str,
"processtypename" type str,
"packtypename" type str,
"sort_emp" type str,
"customerid" type str,
"lvgoodsid" type str,
"dispersesheet" type double,
"dbdeptid" type str,
"dbcustomer" type str,
"dbgulender" type str,
"locked_object" type str,
"locked_date" type DATETIME,
"unlocked_date" type DATETIME,
"preownername" type str,
"ply" type double default value '0',
"tdstate" type str default value '1',
"opselected" type str,
"oplock" type str,
"opinupload" type str,
"opchangeupload" type str,
"opinbatchid" type str,
"opchangebatchid" type str,
"opinupload_date" type DATETIME,
"out_package_status" type str,
"out_package_desc" type str,
"gmpacktype" type str,
"handno" type str,
"saveno" type str,
"drawno" type str,
"dangerouslevel" type str,
"acceptancecriteria" type str,
"shippingmark" type str,
"loclv2" type str,
"payedflag" type str,
"ordergoodsownerid" type str,
"ordergoodsownername" type str
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "NULL";
CREATE PARSER T_GG_SJ_GOODS_parser
TYPE rcd
SCHEMA T_GG_SJ_GOODS_schema;
CREATE TABLE T_GG_SJ_GOODS using T_GG_SJ_GOODS_parser;
CREATE INDEX T_GG_SJ_GOODS_index ON TABLE T_GG_SJ_GOODS (goodsid);<file_sep>/uncomplete/JINSHANG-93/T_HIS_T_STACKING_M.sql
CREATE SCHEMA T_HIS_T_STACKING_M_schema
SOURCE TYPE CSV
FIELDS(
"manu_id" type str,
"stacking_rec_num" type str,
"pick_num" type str,
"order_num" type str,
"company_code" type str,
"ready_num" type str,
"trnp_mode_code" type str,
"carry_company_code" type str,
"carry_company_name" type str,
"n_stacking_rec" type double,
"weight_strc" type double,
"product_dscr" type str,
"shopsign" type str,
"domestic_export" type str,
"storehouse_code" type str,
"lade_code" type str,
"dlv_spot_code" type str,
"private_route_code" type str,
"dlv_spot_name" type str,
"consignee_num" type str,
"consignee_name" type str,
"settle_user_num" type str,
"settle_user_name" type str,
"qlt_decide_code" type str,
"vehicle_code" type str,
"n_grass_pack" type double,
"delivery_date_chr" type str,
"t_delivery" type str,
"dept" type str,
"user_id" type str,
"operator_name" type str,
"product_code_fb1" type str,
"product_code_fb2" type str,
"product_code_fb3" type str,
"expense_status" type str,
"weight_theo" type double,
"weight_type" type str,
"stacking_type" type str,
"code_all_thr_ready" type str,
"stin_sths_code" type str,
"time_receive" type datetime,
"time_proc" type datetime,
"proc_mark" type str,
"error_text1" type str,
"error_text2" type str,
"stacking_print_batch_id" type str,
"ws_flag" type str default value '0',
"ws_date" type datetime,
"seg_no" type str,
"proc_mark_seg" type str,
"product_id" type str,
"wprovider_id" type str,
"insert_time" type datetime,
"downloadflag" type str default value '0',
"optflag" type str default value '0',
"product_adress" type str,
"shopsgin" type str,
"owner_num" type str,
"deptid" type str,
"trans_commission_subid" type str,
"pay_style" type str,
"getflag" type str,
"settle_flag" type str,
"source_seg_no" type str,
"operation_type" type str,
"r_jk_update_date" type datetime
)
SCHEMA DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_HIS_T_STACKING_M_parser
TYPE rcd
SCHEMA T_HIS_T_STACKING_M_schema;
CREATE TABLE T_HIS_T_STACKING_M using T_HIS_T_STACKING_M_parser;
CREATE INDEX T_HIS_T_STACKING_M_index ON TABLE T_HIS_T_STACKING_M (manu_id);
<file_sep>/uncomplete/JINSHANG-74/2_init.sql
create job 1_job(6)
begin
dataset file T_ZY_CK_OUTBILL_1_dataset
(
schema: "T_ZY_CK_OUTBILL_schema",
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_CK_OUTBILL.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc index T_ZY_CK_OUTBILL_index
(
inputs:T_ZY_CK_OUTBILL_1_dataset,
table:T_ZY_CK_OUTBILL
);
dataproc doc T_ZY_CK_OUTBILL_doc
(
inputs:T_ZY_CK_OUTBILL_1_dataset,
table:T_ZY_CK_OUTBILL
);
dataproc map T_ZY_CK_CLEAR_1_map
(
inputs:T_ZY_CK_OUTBILL_1_dataset,
table:T_ZY_CK_OUTBILL
);
end;
run job 1_job (threads:8);
create job 2_job(2)
begin
dataset file T_ZY_GH_CHANGEOWNERLIST_1_dataset
(
schema: "T_ZY_GH_CHANGEOWNERLIST_schema",
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_GH_CHANGEOWNERLIST.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc index T_ZY_GH_CHANGEOWNERLIST_index
(
inputs:T_ZY_GH_CHANGEOWNERLIST_1_dataset,
table:T_ZY_GH_CHANGEOWNERLIST
);
dataproc doc T_ZY_GH_CHANGEOWNERLIST_doc
(
inputs:T_ZY_GH_CHANGEOWNERLIST_1_dataset,
table:T_ZY_GH_CHANGEOWNERLIST
);
dataproc map T_ZY_GH_CHANGEOWNERLIST_1_map
(
inputs:T_ZY_GH_CHANGEOWNERLIST_1_dataset,
table:T_ZY_GH_CHANGEOWNERLIST
);
end;
run job 2_job (threads:8);
create job 3_job(3)
begin
dataset file T_ZY_JG_PROCESS_1_dataset
(
schema: "T_ZY_JG_PROCESS_schema",
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_JG_PROCESS.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc index T_ZY_JG_PROCESS_index
(
inputs:T_ZY_JG_PROCESS_1_dataset,
table:T_ZY_JG_PROCESS
);
dataproc doc T_ZY_JG_PROCESS_doc
(
inputs:T_ZY_JG_PROCESS_1_dataset,
table:T_ZY_JG_PROCESS
);
dataproc map T_ZY_JG_PROCESS_1_map
(
inputs:T_ZY_JG_PROCESS_1_dataset,
table:T_ZY_JG_PROCESS
);
end;
run job 3_job (threads:8);
create job 4_job(4)
begin
dataset file T_XTSZ_BIND_1_dataset
(
schema: "T_XTSZ_BIND_schema",
filename: "/home/zhaoshun/Data/jinshang_New/T_XTSZ_BIND.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc index T_XTSZ_BIND_index
(
inputs:T_XTSZ_BIND_1_dataset,
table:T_XTSZ_BIND
);
dataproc doc T_XTSZ_BIND_doc
(
inputs:T_XTSZ_BIND_1_dataset,
table:T_XTSZ_BIND
);
dataproc map T_XTSZ_BIND_1_map
(
inputs:T_XTSZ_BIND_1_dataset,
table:T_XTSZ_BIND
);
end;
run job 4_job (threads:8);
create job 5_job(3)
begin
dataset file T_XTSZ_BIND_dataset
(
schema: "T_XTSZ_BIND_schema",
filename: "/home/zhaoshun/Data/jinshang_New/T_XTSZ_BIND.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataset file T_GG_SJ_GOODS_dataset
(
schema: "T_GG_SJ_GOODS_schema",
filename: "/home/zhaoshun/Data/jinshang_New/T_GG_SJ_GOODS.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataset file T_ZY_RK_INBILLDETAIL_dataset
(
schema: T_ZY_RK_INBILLDETAIL_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_RK_INBILLDETAIL.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataset file T_ZY_JG_PROCARTICLEDTL_dataset
(
schema: T_ZY_JG_PROCARTICLEDTL_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_JG_PROCARTICLEDTL.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataset file T_ZY_GH_CHANGEOWNERLIST_dataset
(
schema: T_ZY_GH_CHANGEOWNERLIST_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_GH_CHANGEOWNERLIST.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataset file T_ZY_GH_CHANGEOWNERDETAIL_dataset
(
schema: T_ZY_GH_CHANGEOWNERDETAIL_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_GH_CHANGEOWNERDETAIL.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataset file T_ZY_CK_OUTPACK_dataset
(
schema: T_ZY_CK_OUTPACK_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_CK_OUTPACK.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataset file T_ZY_JG_PROCMATERIALDTL_dataset
(
schema: T_ZY_JG_PROCMATERIALDTL_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_JG_PROCMATERIALDTL.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataset file T_ZY_CK_OUTBILL_dataset
(
schema: T_ZY_CK_OUTBILL_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_CK_OUTBILL.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataset file T_ZY_JG_PROCESS_dataset
(
schema: T_ZY_JG_PROCESS_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_JG_PROCESS.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc select T_GG_SJ_GOODS_select
(
fields:
(
(fname:"MANAGENO"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"GRADENUM"),
(fname:"PRODAREANAME"),
(fname:"DEPTID"),
(fname:"NETWEIGHT")
),
inputs:"T_GG_SJ_GOODS_dataset",
order_by:("DEPTID"),
conditions:"GOODSSTATENO = '110'
and DEPT_ID = '1' "
);
dataproc select T_ZY_RK_INBILLDETAIL_select
(
fields:
(
(fname:"MANAGENO"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"GRADENUM"),
(fname:"PRODAREANAME"),
(fname:"DEPTID"),
(fname:"NETWEIGHT")
),
inputs:"T_ZY_RK_INBILLDETAIL_dataset",
order_by:("DEPTID"),
conditions:"TO_CHAR (FIRSTINDATE, '%Y-%m-%d') > '2012-07-16'
and DEPT_ID = '1'"
);
dataproc select T_ZY_GH_CHANGEOWNERDETAIL_select
(
fields:
(
(fname:"MANAGENUM"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"GRADENUM"),
(fname:"PRODAREANAME"),
(fname:"DEPTID"),
(fname:"NETWEIGHT")
),
inputs:"T_ZY_GH_CHANGEOWNERDETAIL_dataset",
order_by:("DEPTID"),
conditions:"vf_DEPTID = '1'
and CHANGEOWNERID = '1'"
);
dataproc select T_ZY_JG_PROCARTICLEDTL_select
(
fields:
(
(fname:"MANAGENO"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"GRADENUM"),
(fname:"PRODAREANAME"),
(fname:"DEPTID"),
(fname:"NETWEIGHT")
),
inputs:"T_ZY_JG_PROCARTICLEDTL_dataset",
order_by:("DEPTID"),
conditions:"DEPTID = '101'
and TO_CHAR (ACCEPTANCE_TIME, '%Y-%m-%d') > '2012-07-16'
and DEPT_ID = '1'"
);
dataproc select T_ZY_CK_OUTPACK_select
(
fields:
(
(fname:"MANAGENUM"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"GRADENUM"),
(fname:"PRODAREANAME"),
(fname:"DEPTID"),
(fname:"NETWEIGHT")
),
inputs:"T_ZY_CK_OUTPACK_dataset",
order_by:("DEPTID"),
conditions:"DEPT_ID = '1'
and vf_DEPTID = '1'"
);
dataproc select T_ZY_GH_CHANGEOWNERDETAIL_2_select
(
fields:
(
(fname:"MANAGENUM"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"GRADENUM"),
(fname:"PRODAREANAME"),
(fname:"DEPTID"),
(fname:"NETWEIGHT")
),
inputs:"T_ZY_GH_CHANGEOWNERDETAIL_dataset",
order_by:("DEPTID"),
conditions:"vf_DEPTID = '1'
and CHANGEOWNERID = '1'"
);
dataproc select T_ZY_JG_PROCMATERIALDTL_select
(
fields:
(
(fname:"MANAGENO"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"GRADENUM"),
(fname:"PRODAREANAME"),
(fname:"DEPTID"),
(fname:"NETWEIGHT")
),
inputs:"T_ZY_JG_PROCMATERIALDTL_dataset",
order_by:("DEPTID"),
conditions:"DEPTID LIKE '080%'
and vf_DEPTID = '1'
and DEPT_ID = '1'"
);
dataproc union union_1_1
(
inputs:("T_GG_SJ_GOODS_select","T_ZY_RK_INBILLDETAIL_select","T_ZY_GH_CHANGEOWNERDETAIL_select","T_ZY_JG_PROCARTICLEDTL_select","T_ZY_CK_OUTPACK_select","T_ZY_GH_CHANGEOWNERDETAIL_2_select","T_ZY_JG_PROCMATERIALDTL_select")
);
dataproc select union_1_select
(
fields:
(
(fname:"MANAGENO"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"GRADENUM"),
(fname:"PRODAREANAME"),
(fname:"DEPTID"),
(fname:"NETWEIGHT")
),
inputs:"union_1_1",
order_by:("MANAGENO")
);
dataproc select union_2_select
(
fields:
(
(fname:"MANAGENO"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"GRADENUM"),
(fname:"PRODAREANAME"),
(fname:"DEPTID"),
(fname:"30",alias:"GOODTYPE",type:"double"),
(fname:"NETWEIGHT")
),
inputs:"union_1_1",
order_by:("MANAGENO")
);
dataproc index T_ZY_CK_OUTBILL_index
(
inputs:union_1_select,
table:union_1
);
dataproc doc T_ZY_CK_OUTBILL_doc
(
inputs:union_1_select,
table:union_1,
format:union_1_parser,
fields:("MANAGENO","KINDNAME","SHOPSIGNNAME","GRADENUM","PRODAREANAME","DEPTID","NETWEIGHT")
);
dataproc select T_ZY_RK_INBILLDETAIL_2_select
(
fields:
(
(fname:"MANAGENO"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"GRADENUM"),
(fname:"PRODAREANAME"),
(fname:"DEPTID"),
(fname:"10",alias:"GOODTYPE",type:"double"),
(fname:"NETWEIGHT")
),
inputs:"T_ZY_RK_INBILLDETAIL_dataset",
order_by:("MANAGENO"),
conditions:"TO_CHAR (FIRSTINDATE, '%Y-%m-%d') >= '2012-07-16'
and TO_CHAR (FIRSTINDATE, '%Y-%m-%d') <= '2015-07-16'
and DEPT_ID = '1'
and OWNER_ID = '1'"
);
dataproc select T_ZY_CK_OUTPACK_2_select
(
fields:
(
(fname:"MANAGENUM"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"GRADENUM"),
(fname:"PRODAREANAME"),
(fname:"DEPTID"),
(fname:"20",alias:"GOODTYPE",type:"double"),
(fname:"NETWEIGHT")
),
inputs:"T_ZY_CK_OUTPACK_dataset",
order_by:("DEPTID"),
conditions:"DEPT_ID = '1'
and OWNER_ID = '1'
and vf_2_DEPTID = '1'
and vf_2_OUTBILLID = '1'"
);
dataproc select T_ZY_GH_CHANGEOWNERDETAIL_3_select
(
fields:
(
(fname:"MANAGENUM"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"GRADENUM"),
(fname:"PRODAREANAME"),
(fname:"DEPTID"),
(fname:"11",alias:"GOODTYPE",type:"double"),
(fname:"NETWEIGHT")
),
inputs:"T_ZY_GH_CHANGEOWNERDETAIL_dataset",
order_by:("DEPTID"),
conditions:"NEWOWNERNO = OWNER_ID
and vf_2_DEPTID = '1'
and vf_2_CHANGEOWNERID = '1'"
);
dataproc select T_ZY_GH_CHANGEOWNERDETAIL_4_select
(
fields:
(
(fname:"MANAGENUM"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"GRADENUM"),
(fname:"PRODAREANAME"),
(fname:"DEPTID"),
(fname:"21",alias:"GOODTYPE",type:"double"),
(fname:"NETWEIGHT")
),
inputs:"T_ZY_GH_CHANGEOWNERDETAIL_dataset",
order_by:("DEPTID"),
conditions:"PREOWNERNO = OWNER_ID
and vf_2_DEPTID = '1'
and vf_2_CHANGEOWNERID = '1'"
);
dataproc select T_ZY_JG_PROCARTICLEDTL_2_select
(
fields:
(
(fname:"MANAGENO"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"GRADENUM"),
(fname:"PRODAREANAME"),
(fname:"DEPTID"),
(fname:"12",alias:"GOODTYPE",type:"double"),
(fname:"NETWEIGHT")
),
inputs:"T_ZY_JG_PROCARTICLEDTL_dataset",
order_by:("DEPTID"),
conditions:"DEPTID LIKE '080%'
and TO_CHAR (ACCEPTANCE_TIME, '%Y-%m-%d') >= '2012-07-16'
and TO_CHAR (ACCEPTANCE_TIME, '%Y-%m-%d') <= '2015-07-16'
and DEPT_ID = '1'
and OWNER_ID = '1'"
);
dataproc select T_ZY_JG_PROCMATERIALDTL_2_select
(
fields:
(
(fname:"MANAGENO"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"GRADENUM"),
(fname:"PRODAREANAME"),
(fname:"DEPTID"),
(fname:"22",alias:"GOODTYPE",type:"double"),
(fname:"NETWEIGHT")
),
inputs:"T_ZY_JG_PROCMATERIALDTL_dataset",
order_by:("DEPTID"),
conditions:"DEPTID LIKE '080%'
and vf_DEPTID = '1'
and vf_PROCESSID = '1'
and vf_2_DEPTID = '1'
and vf_2_PROCESSID = '1'"
);
dataproc union union_all_1
(
inputs:("union_2_select","T_ZY_RK_INBILLDETAIL_2_select","T_ZY_CK_OUTPACK_2_select","T_ZY_GH_CHANGEOWNERDETAIL_3_select","T_ZY_GH_CHANGEOWNERDETAIL_4_select","T_ZY_JG_PROCARTICLEDTL_2_select","T_ZY_JG_PROCMATERIALDTL_2_select")
);
dataproc select union_all_1_select
(
fields:(
(fname:"nvl (MANAGENO,'NULL')",alias:"MANAGENO",type:"string"),
(fname:"nvl (KINDNAME,'NULL')",alias:"KINDNAME",type:"string"),
(fname:"nvl (SHOPSIGNNAME,'NULL')",alias:"SHOPSIGNNAME",type:"string"),
(fname:"nvl (PRODAREANAME,'NULL')",alias:"PRODAREANAME",type:"string"),
(fname:"sum(case GOODTYPE when 10 then NETWEIGHT else 0 end)",alias:"INSTOCK",type:"double"),
(fname:"sum(case GOODTYPE when 20 then NETWEIGHT else 0 end)",alias:"OUTSTOCK",type:"double"),
(fname:"sum(case GOODTYPE when 11 then NETWEIGHT else 0 end)",alias:"CHANGINSTOCK",type:"double"),
(fname:"sum(case GOODTYPE when 21 then NETWEIGHT else 0 end)",alias:"CHANGOUTSTOCK",type:"double"),
(fname:"sum(case GOODTYPE when 12 then NETWEIGHT else 0 end)",alias:"PROCESSINSTOCK",type:"double"),
(fname:"sum(case GOODTYPE when 22 then NETWEIGHT else 0 end)",alias:"PROCESSOUTSTOCK",type:"double"),
(fname:"sum(case GOODTYPE when 30 then NETWEIGHT else 0 end)",alias:"ENDSTOCK",type:"double")
),
inputs: "union_all_1"
);
dataproc select union_all_1_1_select
(
fields:(
(fname:"MANAGENO",type:"string"),
(fname:"KINDNAME",type:"string"),
(fname:"SHOPSIGNNAME",type:"string"),
(fname:"PRODAREANAME",type:"string"),
(fname:"INSTOCK",type:"double"),
(fname:"OUTSTOCK",type:"double"),
(fname:"CHANGINSTOCK",type:"double"),
(fname:"CHANGOUTSTOCK",type:"double"),
(fname:"PROCESSINSTOCK",type:"double"),
(fname:"PROCESSOUTSTOCK",type:"double"),
(fname:"ENDSTOCK",type:"double"),
(fname:"ENDSTOCK-INSTOCK+OUTSTOCK",alias:"BEGINSTOCK",type:"double")
),
inputs: "union_all_1_select",
group_by:(MANAGENO,KINDNAME,SHOPSIGNNAME,PRODAREANAME)
);
dataproc index union_all_2_index
(
inputs:union_all_1_1_select,
table:union_all
);
dataproc doc union_all_2_doc
(
inputs:union_all_1_1_select,
table:union_all,
format:union_all_parser,
fields:("MANAGENO","KINDNAME","SHOPSIGNNAME","PRODAREANAME","INSTOCK","OUTSTOCK","CHANGINSTOCK","CHANGOUTSTOCK","PROCESSINSTOCK","PROCESSOUTSTOCK","ENDSTOCK","BEGINSTOCK")
);
end;
run job 5_job (threads:8);
<file_sep>/complete/JINSHANG-102/job4.sql
create job 4_job(8)
begin
dataset file T_ZY_RK_INBILLDETAIL_dataset
(
schema: T_ZY_RK_INBILLDETAIL_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_RK_INBILLDETAIL.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_RK_INBILLDETAIL_syncher
(
inputs: T_ZY_RK_INBILLDETAIL_dataset,
database:db,
operation:add,
table:T_ZY_RK_INBILLDETAIL,
stream:true,
services :
(
(dataprocname : T_ZY_RK_INBILLDETAIL_dataset,
serviceid: idx_job)
)
);
end;
run job 4_job (threads:8);
<file_sep>/complete/JINSHANG-87/jinshang-87.sql
SELECT sum(weightsum)
FROM t_gu_gub
WHERE R_RECORD_CREATE_DATE>to_date(to_char(sysdate,'yyyy-mm-dd'),'yyyy-mm-dd');
SELECT sum(releaseweight)
FROM t_gu_gub
WHERE R_RECORD_CREATE_DATE>to_date(to_char(sysdate,'yyyy-mm-dd'),'yyyy-mm-dd');
<file_sep>/uncomplete/JINSHANG-81&85/jinshang-81.sql
SELECT T_ZY_CK_OutPack.StockType,
T_ZY_CK_OutBill.OutBillOwnerName OwnerName,
T_ZY_CK_OutPack.ManageNum,
T_ZY_CK_OutPack.OutBillNo BillNo,
SUM(T_ZY_CK_OutPack.NetWeight) NetWeightSum,
SUM(T_ZY_CK_OutPack.GrossWeight) GrossWeightSum,
SUM(T_ZY_CK_OutPack.SheetNum) SheetNumSum,
T_ZY_CK_OutPack.ShopSignName,
T_ZY_CK_OutPack.GradeNum,
T_ZY_CK_OutBill.ForeignListNo,
to_char(T_ZY_CK_OutBill.Outcusttime, 'yyyy-mm-dd') OutBillTime,
T_ZY_CK_OutPack.CreaterName
FROM T_ZY_CK_OutBill
JOIN T_ZY_CK_OutPack ON T_ZY_CK_OutBill.OutBillID = T_ZY_CK_OutPack.OutBillNo
AND T_ZY_CK_OutBill.DeptID = T_ZY_CK_OutPack.DeptID
WHERE T_ZY_CK_OutPack.GoodsStateNo = '40'
AND T_ZY_CK_OutBill.DeptID = '0301'
AND T_ZY_CK_OutBill.ForeignListNo LIKE 'TDGM%'
AND T_ZY_CK_OutPack.ManageNum LIKE 'M%'
AND T_ZY_CK_OutBill.OutBillOwnerNo = 'KH00001'
AND T_ZY_CK_OutBill.Outcusttime >=to_date('2015/7/1','yyyy-mm-dd')
AND T_ZY_CK_OutBill.Outcusttime <to_date('2015/8/1','yyyy-mm-dd')
GROUP BY T_ZY_CK_OutPack.StockType,
T_ZY_CK_OutBill.OutBillOwnerName,
T_ZY_CK_OutPack.ManageNum,
T_ZY_CK_OutPack.OutBillNo,
T_ZY_CK_OutBill.ForeignListNo,
T_ZY_CK_OutBill.Outcusttime,
T_ZY_CK_OutPack.CreaterName,
T_ZY_CK_OutPack.ShopSignName,
T_ZY_CK_OutPack.GradeNum
UNION ALL
SELECT NULL StockType,
t_zy_gh_changeownerlist.proownername OwnerName,
t_zy_gh_changeownerdetail.ManageNum,
t_zy_gh_changeownerdetail.changeownerno BillNo,
SUM(t_zy_gh_changeownerdetail.NetWeight) NetWeightSum,
SUM(t_zy_gh_changeownerdetail.GrossWeight) GrossWeightSum,
SUM(t_zy_gh_changeownerdetail.SheetNum) SheetNumSum,
t_zy_gh_changeownerdetail.ShopSignName,
t_zy_gh_changeownerdetail.GradeNum,
t_zy_gh_changeownerlist.ForeignListNo,
to_char(t_zy_gh_changeownerdetail.incusttime, 'yyyy-mm-dd') OutBillTime,
t_zy_gh_changeownerdetail.r_record_create_user CreaterName
FROM t_zy_gh_changeownerlist
JOIN t_zy_gh_changeownerdetail ON t_zy_gh_changeownerlist.changeownerid = t_zy_gh_changeownerdetail.changeownerno
AND t_zy_gh_changeownerlist.DeptID = t_zy_gh_changeownerdetail.DeptID
WHERE t_zy_gh_changeownerlist.DeptID = '0301'
AND t_zy_gh_changeownerlist.ForeignListNo LIKE 'TDGM%'
AND t_zy_gh_changeownerdetail.ManageNum LIKE 'M%'
AND t_zy_gh_changeownerlist.preownerno = 'KH00001'
AND t_zy_gh_changeownerdetail.incusttime >=to_date('2015/7/1','yyyy-mm-dd')
AND t_zy_gh_changeownerdetail.incusttime <to_date('2015/8/1','yyyy-mm-dd')
GROUP BY t_zy_gh_changeownerlist.proownername,
t_zy_gh_changeownerdetail.ManageNum,
t_zy_gh_changeownerdetail.changeownerno,
t_zy_gh_changeownerdetail.ShopSignName,
t_zy_gh_changeownerdetail.GradeNum,
t_zy_gh_changeownerlist.ForeignListNo,
t_zy_gh_changeownerdetail.incusttime,
t_zy_gh_changeownerdetail.r_record_create_user;
<file_sep>/schema/T_GG_SJ_PRODUCTTYPE.sql
create schema T_GG_SJ_PRODUCTTYPE_schema
source type CSV
fields(
"kindno" type str,
"kindname" type str,
"parentid" type str,
"shortcut" type str,
"remark" type str,
"deptid" type str,
"kindsettleid" type str,
"r_record_create_date" type DATETIME,
"r_record_create_user" type str,
"r_record_update_date" type DATETIME,
"r_record_update_user" type str,
"r_record_is_deleted" type double,
"innercd" type str,
"r_record_change_flag" type double,
"kindcheck" type double,
"kindmemo" type str,
"kindunit" type str,
"kindwei" type double,
"xhunit" type str,
"sheetunit" type str,
"gradenum" type str,
"lv1unit" type str,
"bwlno" type str,
"bwlnoparentid" type str
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "NULL";
CREATE PARSER T_GG_SJ_PRODUCTTYPE_parser
TYPE rcd
SCHEMA T_GG_SJ_PRODUCTTYPE_schema;
CREATE TABLE T_GG_SJ_PRODUCTTYPE using T_GG_SJ_PRODUCTTYPE_parser;
CREATE INDEX T_GG_SJ_PRODUCTTYPE_index ON TABLE T_GG_SJ_PRODUCTTYPE (kindno);<file_sep>/uncomplete/JINSHANG-102/2_init.sql
create job 1_job(1)
begin
dataset file T_ZY_CK_CLEAR_1_dataset
(
schema: "T_ZY_CK_CLEAR_schema",
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_CK_CLEAR.txt",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc index T_ZY_CK_CLEAR_index
(
inputs:T_ZY_CK_CLEAR_1_dataset,
table:T_ZY_CK_CLEAR
);
dataproc doc T_ZY_CK_CLEAR_doc
(
inputs:T_ZY_CK_CLEAR_1_dataset,
table:T_ZY_CK_CLEAR
);
dataproc map T_ZY_CK_CLEAR_1_map
(
inputs:T_ZY_CK_CLEAR_1_dataset,
table:T_ZY_CK_CLEAR
);
end;
run job 1_job (threads:8);
create job 2_job(1)
begin
dataset file T_ZY_JG_PROCESS_1_dataset
(
schema: T_ZY_JG_PROCESS_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_JG_PROCESS.txt",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc index P_index
(
inputs:T_ZY_JG_PROCESS_1_dataset,
table:T_ZY_JG_PROCESS
);
dataproc doc P_doc
(
inputs:T_ZY_JG_PROCESS_1_dataset,
table:T_ZY_JG_PROCESS
);
dataproc map P_1_map
(
inputs:T_ZY_JG_PROCESS_1_dataset,
table:T_ZY_JG_PROCESS
);
end;
run job 2_job (threads:8);
create job 3_job(3)
begin
dataset file T_GG_SJ_GOODS_dataset
(
schema: "T_GG_SJ_GOODS_schema",
filename: "/home/zhaoshun/Data/jinshang_New/T_GG_SJ_GOODS.txt",
serverid: 0,
splitter: (block_size: 10000)
);
dataset file T_ZY_RK_INBILLDETAIL_dataset
(
schema: T_ZY_RK_INBILLDETAIL_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_RK_INBILLDETAIL.txt",
serverid: 0,
splitter: (block_size: 10000)
);
dataset file T_ZY_JG_PROCARTICLEDTL_dataset
(
schema: T_ZY_JG_PROCARTICLEDTL_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_JG_PROCARTICLEDTL.txt",
serverid: 0,
splitter: (block_size: 10000)
);
dataset file T_ZY_GH_CHANGEOWNERLIST_dataset
(
schema: T_ZY_GH_CHANGEOWNERLIST_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_GH_CHANGEOWNERLIST.txt",
serverid: 0,
splitter: (block_size: 10000)
);
dataset file T_ZY_GH_CHANGEOWNERDETAIL_dataset
(
schema: T_ZY_GH_CHANGEOWNERDETAIL_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_GH_CHANGEOWNERDETAIL.txt",
serverid: 0,
splitter: (block_size: 10000)
);
dataset file T_ZY_CK_OUTPACK_dataset
(
schema: T_ZY_CK_OUTPACK_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_CK_OUTPACK.txt",
serverid: 0,
splitter: (block_size: 10000)
);
dataset file T_ZY_JG_PROCMATERIALDTL_dataset
(
schema: T_ZY_JG_PROCMATERIALDTL_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_JG_PROCMATERIALDTL.txt",
serverid: 0,
splitter: (block_size: 10000)
);
dataset file T_ZY_CK_CLEAR_dataset
(
schema: T_ZY_CK_CLEAR_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_CK_CLEAR.txt",
serverid: 0,
splitter: (block_size: 10000)
);
dataset file T_ZY_JG_PROCESS_dataset
(
schema: T_ZY_JG_PROCESS_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_JG_PROCESS.txt",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc select T_ZY_GH_CHANGEOWNERLIST_select
(
fields:
(
(fname:"DEPTID"),
(fname:"CHANGEOWNERID"),
(fname:"NEWOWNERNO"),
(fname:"NEWOWNERNAME"),
(fname:"PREOWNERNO"),
(fname:"PROOWNERNAME"),
(fname:"CHANGEDATE")
),
inputs: "T_ZY_GH_CHANGEOWNERLIST_dataset",
order_by:("DEPTID","CHANGEOWNERID"),
conditions:"DEPTID = '101'
AND CHANGEDATE >= TO_DATE ('2012-07-02', '%Y-%m-%d')"
);
dataproc select T_ZY_GH_CHANGEOWNERDETAIL_select
(
fields:
(
(fname:"PREMANAGENUM"),
(fname:"CASE WHEN ( MANAGENUM IS NULL OR MANAGENUM = '') THEN PREMANAGENUM ELSE MANAGENUM END ",alias:"MANAGENUM",type:"string"),
(fname:"SHOPSIGNID"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREAID"),
(fname:"PRODAREANAME"),
(fname:"GRADENUM"),
(fname:"NETWEIGHT"),
(fname:"GROSSWEIGHT"),
(fname:"SHEETNUM"),
(fname:"FIRSTINDATE"),
(fname:"KINDNAME"),
(fname:"GOODSLOCID"),
(fname:"PACKNUM"),
(fname:"CHANGEOWNERNO"),
(fname:"DEPTID",alias:"DEPTID_1",type:"string"),
(fname:"GRADENUMXH"),
(fname:"SINGLESHEETNUM"),
(fname:"OWNERNAME"),
(fname:"GOODSOWNERMANAGENUM",alias:"GOODSOWNERMANAGENO",type:"string")
),
inputs: "T_ZY_GH_CHANGEOWNERDETAIL_dataset",
order_by:("DEPTID_1","CHANGEOWNERNO")
);
dataproc join T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_join
(
inputs:
(left_input:T_ZY_GH_CHANGEOWNERLIST_select,right_input:T_ZY_GH_CHANGEOWNERDETAIL_select),
join_keys:
(
("left_input.DEPTID","right_input.DEPTID_1"),
("left_input.CHANGEOWNERID","right_input.CHANGEOWNERNO")
)
);
dataproc select T_GG_SJ_GOODS_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"sum(GRADENUMXH)",alias:"GRADENUMXH",type:"double"),
(fname:"sum(SINGLESHEETNUM)",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_GG_SJ_GOODS_dataset",
conditions:"GOODSSTATENO = '110'
AND R_RECORD_IS_DELETED = 0
AND DEPTID = '101'",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE)
);
dataproc select T_ZY_RK_INBILLDETAIL_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"sum(GRADENUMXH)",alias:"GRADENUMXH",type:"double"),
(fname:"sum(SINGLESHEETNUM)",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_ZY_RK_INBILLDETAIL_dataset",
conditions:"DEPTID = '101'
AND GOODSSTATENO = '20'
AND FIRSTINDATE >= TO_DATE ('2012-07-02', '%Y-%m-%d')",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE)
);
dataproc select T_ZY_JG_PROCARTICLEDTL_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"0",alias:"GRADENUMXH",type:"double"),
(fname:"0",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_ZY_JG_PROCARTICLEDTL_dataset",
conditions:"DEPTID = '101'
AND FIRSTINDATE >= TO_DATE ('2012-07-02', '%Y-%m-%d')
AND GOODSSTATENO = '130'",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE)
);
dataproc select T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_select
(
fields:
(
(fname:"NEWOWNERNO",alias:"OWNERID"),
(fname:"NEWOWNERNAME",alias:"OWNERNAME"),
(fname:"MANAGENUM",alias:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"0",alias:"GRADENUMXH",type:"double"),
(fname:"0",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_join",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE)
);
dataproc select T_ZY_CK_OUTPACK_select
(
fields:
(
(fname:"OWNERNO"),
(fname:"OWNERNAME"),
(fname:"MANAGENUM",alias:"MANAGENO"),
(fname:"GOODSOWNERMANAGENUM",alias:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"sum(GRADENUMXH)",alias:"GRADENUMXH",type:"double"),
(fname:"sum(SINGLESHEETNUM)",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_ZY_CK_OUTPACK_dataset",
conditions:"DEPTID = '101'
AND OUTCUSTTIME >= TO_DATE ('2012-07-02', '%Y-%m-%d')",
group_by:(OWNERNO,OWNERNAME,MANAGENUM,GOODSOWNERMANAGENUM,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE)
);
dataproc select T_GG_SJ_GOODS_2_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PACKNUM"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"sum(GRADENUMXH)",alias:"GRADENUMXH",type:"double"),
(fname:"sum(SINGLESHEETNUM)",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_GG_SJ_GOODS_dataset",
conditions:"CREATDATE >= TO_DATE ('2012-07-02', '%Y-%m-%d')",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE)
);
dataproc select T_ZY_JG_PROCMATERIALDTL_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"0",alias:"GRADENUMXH",type:"double"),
(fname:"0",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_ZY_JG_PROCMATERIALDTL_dataset",
conditions:"vf_PROCESSID = '1'
and vf_DEPTID = '1'
and DEPTID = '101' ",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE)
);
dataproc select T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_1_select
(
fields:
(
(fname:"PREOWNERNO",alias:"OWNERID"),
(fname:"PROOWNERNAME",alias:"OWNERNAME"),
(fname:"PREMANAGENUM",alias:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"0",alias:"GRADENUMXH",type:"double"),
(fname:"0",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_join",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE)
);
dataproc union 1_union
(
inputs:("T_GG_SJ_GOODS_select","T_ZY_RK_INBILLDETAIL_select","T_ZY_JG_PROCARTICLEDTL_select","T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_select","T_ZY_CK_OUTPACK_select","T_GG_SJ_GOODS_2_select","T_ZY_JG_PROCMATERIALDTL_select","T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_1_select")
);
dataproc select union_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"sum(GRADENUMXH)",alias:"GRADENUMXH",type:"double"),
(fname:"sum(SINGLESHEETNUM)",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "1_union",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE)
);
dataproc statistics union_all_statistics
(
inputs:union_select,
table:union_all
);
end;
run job 3_job (threads:8);
<file_sep>/schema/T_ZY_CK_CLEAR.sql
CREATE SCHEMA T_ZY_CK_CLEAR_schema
SOURCE TYPE CSV
FIELDS(
"clearno" type str,
"deptid" type str,
"packnum" type str,
"goodsid" type str,
"creatdate" type datetime,
"operater" type str,
"memo" type str,
"r_record_create_date" type datetime,
"r_record_create_user" type str,
"r_record_update_date" type datetime,
"r_record_update_user" type str,
"r_record_is_deleted" type double,
"r_record_change_flag" type double
)
SCHEMA DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_ZY_CK_CLEAR_parser
TYPE rcd
SCHEMA T_ZY_CK_CLEAR_schema;
CREATE TABLE T_ZY_CK_CLEAR using T_ZY_CK_CLEAR_parser;
CREATE INDEX T_ZY_CK_CLEAR_index ON TABLE T_ZY_CK_CLEAR (clearno);
<file_sep>/complete/JINSHANG-102/job5.sql
create job 5_job(8)
begin
dataset file T_ZY_JG_PROCARTICLEDTL_dataset
(
schema: T_ZY_JG_PROCARTICLEDTL_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_JG_PROCARTICLEDTL.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_JG_PROCARTICLEDTL_syncher
(
inputs: T_ZY_JG_PROCARTICLEDTL_dataset,
database:db,
operation:add,
table:T_ZY_JG_PROCARTICLEDTL,
stream:true,
services :
(
(dataprocname : T_ZY_JG_PROCARTICLEDTL_dataset,
serviceid: idx_job)
)
);
end;
run job 5_job (threads:8);
<file_sep>/complete/JINSHANG-76/del.sql
create job t_common_dept_delete_q1(zykie)
begin
dataset file t_common_dept2_dataset
(
schema:T_COMMON_DEPT_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_common_dept/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc syncher t_common_dept_syncher
(
inputs:t_common_dept2_dataset,
database:db,
operator:delete,
table:T_COMMON_DEPT
);
end;
run job t_common_dept_delete_q1(threads:8);
create job t_bm_ui_configure_delete_q1(zykie)
begin
dataset file T_BM_UI_CONFIGURE2_dataset
(
schema:T_BM_UI_CONFIGURE_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_bm_ui_configure/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc syncher t_common_dept_syncher
(
inputs:T_BM_UI_CONFIGURE2_dataset,
database:db,
operator:delete,
table:T_BM_UI_CONFIGURE
);
end;
run job t_bm_ui_configure_delete_q1(threads:8);
create job t_gg_sj_goods_delete_q1(zykie)
begin
dataset file T_GG_SJ_GOODS2_dataset
(
schema:T_GG_SJ_GOODS_schema,
filename:"/home/natt/syncdata/oraclesyncdata/t_gg_sj_goods/delete/delete.csv",
serverid:0,
splitter:(block_size: 10000000)
);
dataproc select T_GG_SJ_GOODS3_select
(
fields:
(
(fname:"nvl (deptid,'NULL')",alias:"deptid",type:"str"),
(fname:"iilmap('T_COMMON_DEPT_map')",alias:"vf_deptname",type:"str"),
(fname:"nvl (gradenum, 'NULL')",alias:"gradenum",type:"str"),
(fname:"nvl (shopsignid, 'NULL')",alias:"shopsignid",type:"str"),
(fname:"nvl (shopsignname, 'NULL')",alias:"shopsignname",type:"str"),
(fname:"nvl (prodareaid, 'NULL')",alias:"prodareaid",type:"str"),
(fname:"nvl (prodareaname, 'NULL')",alias:"prodareaname",type:"str"),
(fname:"nvl (kindno, 'NULL')",alias:"kindno",type:"str"),
(fname:"nvl (kindname, 'NULL')",alias:"kindname",type:"str"),
(fname:"nvl (ownername, 'NULL')",alias:"ownername",type:"str"),
(fname:"nvl (goodsownerpackno, 'NULL')",alias:"goodsownerpackno",type:"str"),
(fname:"nvl (netweight, 0)",alias:"netweight",type:"str"),
(fname:"nvl (grossweight, 0)",alias:"grossweight",type:"str"),
(fname:"nvl (sheetnum, 0)",alias:"sheetnum",type:"str"),
(fname:"iilmap('T_BM_UI_CONFIGURE_map',1)",alias:"vf_count",type:"str")
),
inputs: "T_GG_SJ_GOODS2_dataset"
);
dataproc select T_GG_SJ_GOODS11_select
(
fields:
(
(fname:"deptid",type:"str"),
(fname:"vf_deptname",type:"str"),
(fname:"gradenum",type:"str"),
(fname:"shopsignid",type:"str"),
(fname:"shopsignname",type:"str"),
(fname:"prodareaid",type:"str"),
(fname:"prodareaname",type:"str"),
(fname:"kindno",type:"str"),
(fname:"kindname",type:"str"),
(fname:"ownername",type:"str"),
(fname:"goodsownerpackno",type:"str"),
(fname:"sum(netweight)",alias:"sumnetweight",type:"str"),
(fname:"sum(grossweight)",alias:"sumgrossweight",type:"str"),
(fname:"sum(sheetnum)",alias:"sumsheetnum",type:"str"),
(fname:"vf_count",type:"str"),
(fname:"case vf_count when '0' then '101%' else '101' end",alias:"vf_countcase",type:"str")
),
inputs: "T_GG_SJ_GOODS3_select",
group_by:(deptid,vf_deptname,gradenum,shopsignid,shopsignname,prodareaid,prodareaname,kindno,kindname,ownername,goodsownerpackno,vf_count,vf_countcase)
);
dataproc syncher T_GG_SJ_GOODS1_syncher
(
inputs: T_GG_SJ_GOODS11_select,
database:db,
operator:delete,
table:T_GG_SJ_GOODS1
);
end;
run job t_gg_sj_goods_delete_q1(threads:8);<file_sep>/complete/JINSHANG-102/job6.sql
create job 6_job(8)
begin
dataset file T_ZY_GH_CHANGEOWNERLIST_dataset
(
schema: T_ZY_GH_CHANGEOWNERLIST_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_GH_CHANGEOWNERLIST.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_GH_CHANGEOWNERLIST_syncher
(
inputs: T_ZY_GH_CHANGEOWNERLIST_dataset,
database:db,
operation:add,
table:T_ZY_GH_CHANGEOWNERLIST,
stream:true,
services :
(
(dataprocname : T_ZY_GH_CHANGEOWNERLIST_dataset,
serviceid: idx_job)
)
);
end;
run job 6_job (threads:8);
<file_sep>/uncomplete/JINSHANG-100/1_schema.sql
create database db;
use db;
create schema T_GG_SJ_GOODS_schema
source type CSV
fields(
"GOODSID" type string,
"DEPTID" type string,
"PACKNUM" type string,
"BARCODE" type string,
"GOODSLOCID" type string,
"STOCKTYPE" type string,
"OWNERID" type string,
"OWNERNAME" type string,
"GOODSOWNERMANAGENO" type string,
"INBILLID" type string,
"GOODSOWNERPACKNO" type string,
"MANAGENO" type string,
"FEEBEGINDATE" type DATETIME,
"CONTRACTID" type string,
"GOODSSTATENO" type string,
"GOODSSTATENAME" type string,
"CHANGEOWNERTIMES" type double default value '0',
"GOODSTYPE" type string,
"GOODSTYPENAME" type string,
"KINDNO" type string,
"KINDNAME" type string,
"SHOPSIGNID" type string,
"SHOPSIGNNAME" type string,
"PRODAREAID" type string,
"PRODAREANAME" type string,
"GOODSBATCHNO" type string,
"PRODSTOVENO" type string,
"GRADENUM" type string,
"GRADENUMTHICK" type double,
"GRADENUMWIDTH" type double,
"GRADENUMLENGTH" type double,
"GROSSWEIGHT" type double,
"NETWEIGHT" type double,
"POUNDWEIGHT" type double,
"CHECKWEIGHT" type double,
"SHEETNUM" type double default value '1',
"UNIT" type string,
"PACKTYPE" type string,
"STOCKAREAID" type string,
"QUALITYMEMO" type string,
"FIRSTINOWNERID" type string,
"FIRSTINDATE" type datetime,
"OUTBILLID" type string,
"LDGLISTID" type string,
"MATERIALGOODSID" type string,
"INTYPE" type string,
"OUTTYPE" type string,
"BILLSTATE" type double,
"OUTBILLTIME" type DATETIME,
"MEMO" type string,
"EXTSYSPLANOBJECTID" type string,
"EXTSYSKINDID" type string,
"EXTSYSSHOPSIGNID" type string,
"EXTSYSPRODAREAID" type string,
"FOREIGNLISTNO" type string,
"SENDFLAG" type double,
"R_RECORD_CREATE_DATE" type DATETIME,
"R_RECORD_CREATE_USER" type string,
"R_RECORD_UPDATE_DATE" type DATETIME,
"R_RECORD_UPDATE_USER" type string,
"R_RECORD_IS_DELETED" type double,
"INCUSTTIME" type DATETIME,
"SIDEMARK" type string,
"TEMP" type string,
"LENGTH_PRODUCT_MAX" type double,
"HEAT_NUM" type string,
"TEST_LOT_NUM" type string,
"R_RECORD_CHANGE_FLAG" type double,
"SDPRICE" type string,
"EXTSYSGRADENUM" type string,
"COLLECTIONMANNAME" type string,
"LOCKFLAG" type string,
"CHECK_TYPE" type string,
"OUTDATE" type DATETIME,
"YFWEIGHT" type double,
"YFSHEET" type double,
"PREGOODSID" type string,
"ISSPLIT" type double,
"GRADENUMXH" type double,
"GRADENUMWHDW" type string,
"SPLITTIME" type double,
"ISCLEAR" type double,
"SINGLESHEETNUM" type double,
"GRADENUMHZ" type string,
"GRADENUMTHICKHZ" type double,
"SINGLESHEET" type double,
"LOCLV1" type string,
"SINGLESHEETUNIT" type string,
"DCEMP" type string,
"QZEMP" type string,
"DCEMP2" type string,
"SHIPNO" type string,
"SHIPNAME" type string,
"SHIPCD" type string,
"SHIPLEVEL" type string,
"CMMEMO" type string,
"CMMEMOCK" type string,
"SHOPSIGNCD" type string,
"PROCESSTYPENAME" type string,
"PACKTYPENAME" type string,
"SORT_EMP" type string,
"CUSTOMERID" type string,
"LVGOODSID" type string,
"DISPERSESHEET" type double,
"DBDEPTID" type string,
"DBCUSTOMER" type string,
"DBGULENDER" type string,
"LOCKED_OBJECT" type string,
"LOCKED_DATE" type DATETIME,
"UNLOCKED_DATE" type DATETIME,
"PREOWNERNAME" type string,
"PLY" type double default value '0',
"TDSTATE" type string default value '1',
"OPSELECTED" type string,
"OPLOCK" type string,
"OPINUPLOAD" type string,
"OPCHANGEUPLOAD" type string,
"OPINBATCHID" type string,
"OPCHANGEBATCHID" type string,
"OPINUPLOAD_DATE" type DATETIME,
"OUT_PACKAGE_STATUS" type string,
"OUT_PACKAGE_DESC" type string,
"GMPACKTYPE" type string,
"HANDNO" type string,
"SAVENO" type string,
"DRAWNO" type string,
"DANGEROUSLEVEL" type string,
"ACCEPTANCECRITERIA" type string,
"SHIPPINGMARK" type string,
"LOCLV2" type string,
"PAYEDFLAG" type string,
"ORDERGOODSOWNERID" type string,
"ORDERGOODSOWNERNAME" type string,
"CREATDATE" type `expr` data type datetime value iilmap("T_ZY_CK_CLEAR_map",GOODSID)
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_GG_SJ_GOODS_parser
TYPE rcd
SCHEMA T_GG_SJ_GOODS_schema;
CREATE TABLE T_GG_SJ_GOODS using T_GG_SJ_GOODS_parser;
create schema T_ZY_RK_INBILLDETAIL_schema
source type CSV
fields(
"GOODSID" type string not null,
"DEPTID" type string not null,
"PACKNUM" type string,
"BARCODE" type string,
"GOODSLOCID" type string,
"STOCKTYPE" type string,
"OWNERID" type string,
"OWNERNAME" type string,
"GOODSOWNERMANAGENO" type string,
"INBILLID" type string,
"GOODSOWNERPACKNO" type string,
"MANAGENO" type string not null,
"FEEBEGINDATE" type datetime,
"CONTRACTID" type string,
"GOODSSTATENO" type string,
"GOODSSTATENAME" type string,
"CHANGEOWNERTIMES" type double,
"GOODSTYPE" type string,
"GOODSTYPENAME" type string,
"KINDNO" type string,
"KINDNAME" type string,
"SHOPSIGNID" type string,
"SHOPSIGNNAME" type string,
"PRODAREAID" type string,
"PRODAREANAME" type string,
"GOODSBATCHNO" type string,
"PRODSTOVENO" type string,
"GRADENUM" type string,
"GRADENUMTHICK" type double,
"GRADENUMWIDTH" type double,
"GRADENUMLENGTH" type double,
"GROSSWEIGHT" type double,
"NETWEIGHT" type double,
"POUNDWEIGHT" type double,
"CHECKWEIGHT" type double,
"SHEETNUM" type double,
"UNIT" type string,
"STOCKAREAID" type string,
"QUALITYMEMO" type string,
"FIRSTINOWNERID" type string,
"FIRSTINDATE" type datetime,
"OUTBILLID" type string,
"LDGLISTID" type string,
"MATERIALGOODSID" type string,
"INTYPE" type string,
"OUTTYPE" type string,
"BILLSTATE" type double,
"OUTBILLTIME" type datetime,
"MEMO" type string,
"EXTSYSPLANOBJECTID" type string,
"EXTSYSKINDID" type string,
"EXTSYSSHOPSIGNID" type string,
"EXTSYSPRODAREAID" type string,
"SENDFLAG" type double,
"R_RECORD_CREATE_DATE" type datetime,
"R_RECORD_CREATE_USER" type string,
"R_RECORD_UPDATE_DATE" type datetime,
"R_RECORD_UPDATE_USER" type string,
"R_RECORD_IS_DELETED" type double,
"INCUSTTIME" type datetime,
"FOREIGNLISTNO" type string,
"SIDEMARK" type string,
"LENGTH_PRODUCT_MAX" type double,
"R_RECORD_CHANGE_FLAG" type double,
"SDPRICE" type string,
"INBILLEMPNAME" type string,
"EXTSYSGRADENUM" type string,
"HEAT_NUM" type string,
"ERRORMESSAGE" type string,
"CHECK_TYPE" type string,
"OUTDATE" type datetime,
"YFWEIGHT" type double,
"YFSHEET" type double,
"PREGOODSID" type string,
"SINGLESHEETNUM" type double,
"GRADENUMHZ" type string,
"GRADENUMTHICKHZ" type double,
"SINGLESHEET" type double,
"GRADENUMXH" type double,
"SHIPNO" type string,
"SHIPNAME" type string,
"SHIPCD" type string,
"SHIPLEVEL" type string,
"SORT_EMP" type string,
"DBRKFLAG" type double,
"DBCKFLAG" type double,
"DBOWNER" type string,
"DBGOODSID" type string,
"DISPERSESHEET" type double,
"PLY" type double,
"OUT_PACKAGE_STATUS" type string,
"OUT_PACKAGE_DESC" type string,
"GMPACKTYPE" type string,
"HANDNO" type string,
"SAVENO" type string,
"DANGEROUSLEVEL" type string,
"ACCEPTANCECRITERIA" type string,
"SHIPPINGMARK" type string,
"GRADENUMWHDW" type string,
"LOCLV2" type string,
"PACKTYPE" type string,
"PAYEDFLAG" type string,
"COLLECTIONMANNAME" type string,
"LOCLV1" type string
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_ZY_RK_INBILLDETAIL_parser
TYPE rcd
SCHEMA T_ZY_RK_INBILLDETAIL_schema;
CREATE TABLE T_ZY_RK_INBILLDETAIL using T_ZY_RK_INBILLDETAIL_parser;
create schema T_ZY_JG_PROCARTICLEDTL_schema
source type CSV
fields(
"PROCARTICLEDTLID" type string not null,
"DEPTID" type string not null,
"PROCESSID" type string,
"PACKNUM" type string,
"BARCODE" type string,
"GOODSLOCID" type string,
"OWNERID" type string,
"OWNERNAME" type string,
"GOODSOWNERMANAGENO" type string,
"GOODSOWNERPACKNO" type string,
"MANAGENO" type string not null,
"FEEBEGINDATE" type datetime,
"CONTRACTID" type string,
"GOODSSTATENO" type string,
"GOODSSTATENAME" type string,
"CHANGEOWNERTIMES" type double,
"GOODSTYPE" type string,
"GOODSTYPENAME" type string,
"KINDNO" type string,
"KINDNAME" type string,
"SHOPSIGNID" type string,
"SHOPSIGNNAME" type string,
"PRODAREAID" type string,
"PRODAREANAME" type string,
"GOODSBATCHNO" type string,
"PRODSTOVENO" type string,
"GRADENUM" type string,
"GRADENUMTHICK" type double,
"GRADENUMWIDTH" type double,
"GRADENUMLENGTH" type double,
"GROSSWEIGHT" type double,
"NETWEIGHT" type double,
"POUNDWEIGHT" type double,
"CHECKWEIGHT" type double,
"SHEETNUM" type double,
"PACKTYPE" type string,
"PACKTYPENAME" type string,
"UNIT" type string,
"QUALITYMEMO" type string,
"FIRSTINOWNERID" type string,
"FIRSTINDATE" type datetime,
"PRINTFLAG" type string,
"QUALITY" type string,
"QAMEMBER" type string,
"QAFLAG" type string,
"PROCESSQUALITYID" type string,
"R_RECORD_CREATE_DATE" type datetime,
"R_RECORD_CREATE_USER" type string,
"R_RECORD_UPDATE_DATE" type datetime,
"R_RECORD_UPDATE_USER" type string,
"R_RECORD_IS_DELETED" type double,
"ORDERBY" type double,
"R_RECORD_CHANGE_FLAG" type double,
"INSTRUCTION_ID" type string,
"SENDFLAG" type double,
"ACCEPTANCE_TIMES" type double,
"ACCEPTANCER" type string,
"ACCEPTANCE_TIME" type datetime,
"SKINWEIGHT" type double,
"CYYWEIGHT" type double,
"GOODSID" type string,
"ARTICLEMEMO1" type string,
"ARTICLEMEMO2" type string,
"ARTICLEMEMO3" type string,
"ARTICLEMEMO4" type string,
"ARTICLEMEMO5" type string,
"ARTICLEMEMO6" type string,
"ARTICLEMEMO7" type string,
"ARTICLEMEMO8" type string,
"ARTICLEMEMO9" type string,
"ARTICLEMEMO10" type string,
"ARTICLEYS" type string,
"CUSTOMERID" type string,
"CBXJGTYPE" type string,
"WALLWIDTH" type double,
"WOODTYPE" type string,
"WOODTYPENAME" type string,
"CHANXIAN" type string,
"DRAWNO" type string,
"ZCFLAG" type string ,
"CLASSGROUP" type string,
"LOCLV1" type string,
"LOCLV2" type string
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_ZY_JG_PROCARTICLEDTL_parser
TYPE rcd
SCHEMA T_ZY_JG_PROCARTICLEDTL_schema;
CREATE TABLE T_ZY_JG_PROCARTICLEDTL using T_ZY_JG_PROCARTICLEDTL_parser;
create schema T_ZY_GH_CHANGEOWNERLIST_schema
source type CSV
fields(
"CHANGEOWNERID" type string not null,
"DEPTID" type string not null,
"PROOWNERNAME" type string,
"PREOWNERNO" type string,
"CONTRACTNO" type string,
"PREMANAGENUM" type string,
"NEWOWNERNAME" type string,
"NEWOWNERNO" type string,
"NEWMANAGENUM" type string,
"CHANGEDATE" type datetime,
"GETBIILLDATE" type datetime,
"FOREIGNLISTNO" type string,
"ORDERLISTNO" type string,
"OUTCARBILLTIME" type datetime,
"OUTCARBILLEMPNO" type string,
"OUTCARBILLEMPNAME" type string,
"OUTCARBILLMEMO" type string,
"R_RECORD_CREATE_DATE" type datetime,
"R_RECORD_CREATE_USER" type string,
"R_RECORD_UPDATE_DATE" type datetime,
"R_RECORD_UPDATE_USER" type string,
"R_RECORD_IS_DELETED" type double,
"NETWEIGHTSUM" type double,
"GROSSWEIGHTSUM" type double,
"SHEETNUMSUM" type double,
"ORIGINSETTLE" type double,
"R_RECORD_CHANGE_FLAG" type double,
"ISSPLIT" type double,
"OUTPASSWORD" type string,
"INVOICEKINDID" type string,
"INVOICEKINDNAME" type string,
"SORTEMP" type string,
"SORTEMPTELE" type string,
"SETTLESTATUS" type string,
"CONFIRM_DATE" type datetime,
"CONFIRM_EMP" type string,
"CONFIRM_FLAG" type string,
"DTLCOUNT" type double,
"ISSETTLED" type double,
"JFSENDFLAG" type double,
"JYZXFLAG" type double,
"SENDMESSAGE" type string,
"JYZXCHECKDATE" type datetime,
"JYZXCHECKFLAG" type double,
"IDCODE" type string,
"USERNAME" type string
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_ZY_GH_CHANGEOWNERLIST_parser
TYPE rcd
SCHEMA T_ZY_GH_CHANGEOWNERLIST_schema;
CREATE TABLE T_ZY_GH_CHANGEOWNERLIST using T_ZY_GH_CHANGEOWNERLIST_parser;
create schema T_ZY_GH_CHANGEOWNERDETAIL_schema
source type CSV
fields(
"MANAGENO" type string not null,
"DEPTID" type string not null,
"CHANGEOWNERNO" type string,
"PACKNUM" type string,
"GOODSID" type string,
"INBEGINTIME" type datetime,
"BARCODE" type string,
"GOODSLOCID" type string,
"OWNERNAME" type string,
"OWNERNO" type string,
"PREGOODSOWNERMANAGENUM" type string,
"GOODSOWNERMANAGENUM" type string,
"GOODSOWNERPACKNUM" type string,
"PREMANAGENUM" type string,
"MANAGENUM" type string,
"CONTRACTNO" type string,
"GOODSSTATENAME" type string,
"GOODSSTATENO" type string,
"GOODSTYPENAME" type string,
"GOODSTYPE" type string,
"KINDNAME" type string,
"KINDNO" type string,
"SHOPSIGNNAME" type string,
"SHOPSIGNID" type string,
"PRODAREANAME" type string,
"PRODAREAID" type string,
"GOODSBATCHNUM" type string,
"GRADENUM" type string,
"GRADENUMTHICK" type double,
"GRADENUMWIDTH" type double,
"GRADENUMLENGTH" type double,
"GROSSWEIGHT" type double,
"NETWEIGHT" type double,
"POUNDWEIGHT" type double,
"CHECKWEIGHT" type double,
"SHEETNUM" type double,
"QUALITYMEMO" type string,
"FIRSTINOWNERID" type string,
"FIRSTINDATE" type datetime,
"INBILLID" type string,
"INTYPE" type string,
"PREMEMO" type string,
"MEMO" type string,
"FOREIGNLISTNO" type string,
"PREFOREIGNLISTNO" type string,
"ORDERLISTNO" type string,
"PREORDERLISTNO" type string,
"R_RECORD_CREATE_DATE" type datetime,
"R_RECORD_CREATE_USER" type string,
"R_RECORD_UPDATE_DATE" type datetime,
"R_RECORD_UPDATE_USER" type string,
"R_RECORD_IS_DELETED" type double,
"PREINCUSTTIME" type datetime,
"INCUSTTIME" type datetime,
"SENDFLAG" type double,
"OUTCHANGESENDFLAG" type double,
"CHANGETIMES" type double,
"ORIGINOWNERNAME" type string,
"ORIGINOWNERNO" type string,
"R_RECORD_CHANGE_FLAG" type double,
"CUSTDAY" type double,
"SDPRICE" type string,
"NEWDATE" type datetime,
"PREGOODSID" type string,
"CHECK_TYPE" type string,
"SINGLESHEETNUM" type double,
"GRADENUMXH" type double,
"SIDEMARK" type string,
"SORT_EMP" type string,
"DISPERSESHEET" type double,
"PLY" type double ,
"ORIGINSHEETNUM" type double,
"ORIGINNETWEIGHT" type double,
"ISSPLIT" type double,
"GMPACKTYPE" type string,
"PREFOREIGNLISTCODE" type string,
"CONFIRMFLAG" type string ,
"JYZXFLAG" type double,
"LOCLV1" type string,
"LOCLV2" type string,
"OUTNETWEIGHT" type double
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_ZY_GH_CHANGEOWNERDETAIL_parser
TYPE rcd
SCHEMA T_ZY_GH_CHANGEOWNERDETAIL_schema;
CREATE TABLE T_ZY_GH_CHANGEOWNERDETAIL using T_ZY_GH_CHANGEOWNERDETAIL_parser;
create schema T_ZY_CK_OUTPACK_schema
source type CSV
fields(
"OUTPACKID" type string not null,
"DEPTID" type string not null,
"OUTBILLNO" type string not null,
"GOODSID" type string,
"PACKNUM" type string,
"BARCODE" type string,
"GOODSLOCID" type string,
"OWNERNAME" type string,
"OWNERNO" type string,
"GOODSOWNERMANAGENUM" type string,
"GOODSOWNERPACKNUM" type string,
"MANAGENUM" type string,
"INBEGINTIME" type datetime,
"CONTRACTNO" type string,
"OLDGOODSSTATENAME" type string,
"OLDGOODSSTATENO" type string,
"GOODSSTATENAME" type string,
"GOODSSTATENO" type string,
"GOODSTYPENAME" type string,
"GOODSTYPE" type string,
"KINDNAME" type string,
"KINDNO" type string,
"SHOPSIGNNAME" type string,
"SHOPSIGNID" type string,
"PRODAREANAME" type string,
"PRODAREAID" type string,
"GOODSBATCHNUM" type string,
"GRADENUM" type string,
"GRADENUMTHICK" type double,
"GRADENUMWIDTH" type double,
"GRADENUMLENGTH" type double,
"GROSSWEIGHT" type double,
"OUTGROSSWEIGHT" type double,
"OUTNETWEIGHT" type double,
"NETWEIGHT" type double,
"POUNDWEIGHT" type double,
"CHECKWEIGHT" type double,
"SHEETNUM" type double,
"PACKTYPE" type string,
"QUALITYMEMO" type string,
"FIRSTINOWNERID" type string,
"FIRSTINDATE" type datetime,
"INBILLID" type string,
"MATERIALGOODSID" type string,
"INTYPE" type string,
"MEMO" type string,
"CUSTBILL" type string,
"SENDFLAG" type double,
"VELFLAG" type double,
"R_RECORD_CREATE_DATE" type datetime,
"R_RECORD_CREATE_USER" type string,
"R_RECORD_UPDATE_DATE" type datetime,
"R_RECORD_UPDATE_USER" type string,
"R_RECORD_IS_DELETED" type double,
"FOREIGNLISTNO" type string,
"CREATEID" type string,
"CREATERNAME" type string,
"SIDEMARK" type string,
"STOCKTYPE" type string,
"OUTBILLPICKOWNERNAME" type string,
"R_RECORD_CHANGE_FLAG" type double,
"INCUSTTIME" type datetime,
"CUSTDAY" type double,
"SDPRICE" type string,
"OUTTYPE" type string,
"EXTSYSKINDID" type string,
"EXTSYSSHOPSIGNID" type string,
"EXTSYSGRADENUM" type string,
"PRODSTOVENO" type string,
"CHECK_TYPE" type string,
"SINGLESHEETNUM" type double,
"SINGLESHEET" type double,
"GRADENUMXH" type double,
"OUTBILLPICKOWNERNO" type string,
"OUTPASSWORD" type string,
"SHIPNO" type string,
"SHIPNAME" type string,
"SHIPCD" type string,
"SHIPLEVEL" type string,
"SORT_EMP" type string,
"LENGTH_PRODUCT_MAX" type double,
"PACKTYPENAME" type string,
"DBCKFLAG" type double,
"DBOWNER" type string,
"DISPERSESHEET" type double,
"OUTCARNO" type string,
"OUTCUSTTIME" type datetime,
"PLY" type double,
"ORIGINOUTSHEETNUM" type double,
"OUTSHEETNUM" type double,
"ORIGINOUTNETWEIGHT" type double,
"ISSPLIT" type double,
"PREFOREIGNLISTNO" type string,
"DRAWNO" type string,
"HANDNO" type string,
"SAVENO" type string,
"LOCLV2" type string,
"GRADENUMWHDW" type string,
"OPTFLAG" type double,
"PAYEDFLAG" type string,
"SALE_PRICE_AT" type double,
"PRICE" type double,
"CHANGEOWNERTIMES" type double,
"CONTRACT_ID" type string,
"CONTRACT_SUBID" type string,
"JYZXFLAG" type double,
"OUTBILLDATE" type datetime,
"ROLLPLY" type double,
"READYOUT" type datetime,
"VALIDDATE" type datetime,
"LOCKCOUNT" type double ,
"LOCLV1" type string
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_ZY_CK_OUTPACK_parser
TYPE rcd
SCHEMA T_ZY_CK_OUTPACK_schema;
CREATE TABLE T_ZY_CK_OUTPACK using T_ZY_CK_OUTPACK_parser;
create schema T_ZY_CK_CLEAR_schema
source type CSV
fields(
"CLEARNO" type string,
"DEPTID" type string,
"PACKNUM" type string,
"GOODSID" type string not null,
"CREATDATE" type datetime,
"OPERATER" type string,
"MEMO" type string,
"R_RECORD_CREATE_DATE" type datetime,
"R_RECORD_CREATE_USER" type string,
"R_RECORD_UPDATE_DATE" type datetime,
"R_RECORD_UPDATE_USER" type string,
"R_RECORD_IS_DELETED" type double,
"R_RECORD_CHANGE_FLAG" type double
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_ZY_CK_CLEAR_parser
TYPE rcd
SCHEMA T_ZY_CK_CLEAR_schema;
CREATE TABLE T_ZY_CK_CLEAR using T_ZY_CK_CLEAR_parser;
create index T_ZY_CK_CLEAR_index on table T_ZY_CK_CLEAR(GOODSID);
create map T_ZY_CK_CLEAR_map on table T_ZY_CK_CLEAR
KEY (GOODSID)
VALUE (CREATDATE)
TYPE datetime
WHERE CREATDATE >= TO_DATE ('2012-07-02', '%Y-%m-%d');
create schema T_ZY_JG_PROCMATERIALDTL_schema
source type CSV
fields(
"PROCMATERIALDTLID" type string not null,
"DEPTID" type string not null,
"PROCESSID" type string,
"PACKNUM" type string,
"GOODSID" type string,
"BARCODE" type string,
"GOODSLOCID" type string,
"OWNERID" type string,
"OWNERNAME" type string,
"GOODSOWNERMANAGENO" type string,
"GOODSOWNERPACKNO" type string,
"MANAGENO" type string,
"FEEBEGINDATE" type datetime,
"CONTRACTID" type string,
"GOODSSTATENO" type string,
"GOODSSTATENAME" type string,
"CHANGEOWNERTIMES" type double,
"GOODSTYPE" type string,
"GOODSTYPENAME" type string,
"KINDNO" type string,
"KINDNAME" type string,
"SHOPSIGNID" type string,
"SHOPSIGNNAME" type string,
"PRODAREAID" type string,
"PRODAREANAME" type string,
"GOODSBATCHNO" type string,
"PRODSTOVENO" type string,
"GRADENUM" type string,
"GRADENUMTHICK" type double,
"GRADENUMWIDTH" type double,
"GRADENUMLENGTH" type double,
"GROSSWEIGHT" type double,
"NETWEIGHT" type double,
"POUNDWEIGHT" type double,
"CHECKWEIGHT" type double,
"SHEETNUM" type double,
"PACKTYPE" type string,
"PACKTYPENAME" type string,
"UNIT" type string,
"QUALITYMEMO" type string,
"FIRSTINOWNERID" type string,
"FIRSTINDATE" type datetime,
"INBILLID" type string,
"INTYPE" type string,
"R_RECORD_CREATE_DATE" type datetime,
"R_RECORD_CREATE_USER" type string,
"R_RECORD_UPDATE_DATE" type datetime,
"R_RECORD_UPDATE_USER" type string,
"R_RECORD_IS_DELETED" type double,
"ORDERBY" type double,
"R_RECORD_CHANGE_FLAG" type double,
"SHOPSIGNCD" type string,
"CUSTOMERID" type string,
"CHANXIAN" type string,
"PLY" type double,
"MEMO" type string,
"JYZXFLAG" type double,
"LOCLV1" type string,
"LOCLV2" type string,
"SPECIALMEMO" type string,
"vf_PROCESSID" type `expr` data type string value iilmap("PROCESSID_map",PROCESSID),
"vf_DEPTID" type `expr` data type string value iilmap("DEPTID_map",DEPTID)
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_ZY_JG_PROCMATERIALDTL_parser
TYPE rcd
SCHEMA T_ZY_JG_PROCMATERIALDTL_schema;
CREATE TABLE T_ZY_JG_PROCMATERIALDTL using T_ZY_JG_PROCMATERIALDTL_parser;
create schema T_ZY_JG_PROCESS_schema
source type CSV
fields(
"PROCESSID" type string not null,
"DEPTID" type string,
"CUSTOMERID" type string,
"PROCESSDEPT" type string,
"PROCESSDEPTNAME" type string,
"PROCESSTYPE" type string,
"PROCESSTYPENAME" type string,
"PROCESSMOLD" type string,
"PROCESSMOLDNAME" type string,
"PROCESSSTATE" type double,
"PLANENDDATE" type datetime,
"KINDNO" type string,
"KINDNAME" type string,
"PACKTYPE" type string,
"PACKTYPENAME" type string,
"WOODTYPE" type string,
"WOODTYPENAME" type string,
"STRAPTYPE" type string,
"STRAPTYPENAME" type string,
"PROCESSPRECISION" type string,
"ORDERINFO" type string,
"FEE" type double,
"LENGTH" type double,
"WEIGTHT" type double,
"SHEET" type double,
"PIECENUM" type double,
"OUTCARBILLTIME" type datetime,
"OUTCARBILLEMPNO" type string,
"OUTCARBILLEMPNAME" type string,
"PLANNO" type string,
"PREWEIGHTSUM" type double,
"MENO" type string,
"GRADENUMRANGE" type string,
"GRADENUMRANGENAME" type string,
"THICKRANGE" type string,
"THICKRANGENAME" type string,
"R_RECORD_CREATE_DATE" type datetime,
"R_RECORD_CREATE_USER" type string,
"R_RECORD_UPDATE_DATE" type datetime,
"R_RECORD_UPDATE_USER" type string,
"R_RECORD_IS_DELETED" type double,
"R_RECORD_CHANGE_FLAG" type double,
"DENSITY" type double,
"PROCESSCUSTOMNO" type string,
"PROCESSCUSTOMNAME" type string,
"SETTLEDEPTNO" type string,
"SETTLEDEPTNAME" type string,
"SETTLE_STAUS" type string,
"SETTLE_STAUS_NAME" type string,
"SETTLE_NO" type string,
"SETTLE_DATE" type datetime,
"ACCEPTANCER" type string,
"ACCEPTANCE_TIME" type datetime,
"CHANXIAN" type string,
"MDDEPTNAME" type string,
"ARTICLEYS" type string,
"MATERIALGRADENUM" type string,
"MATERIALSHOPSIGN" type string,
"CBXJGTYPE" type string,
"JGMESSAGE" type string,
"JGSTATUS" type string,
"ARTICLEFEE" type string,
"ISSETTLED" type double,
"SPECIALDOWN" type string,
"ARTICLESHEET" type double,
"ARTICLEWEIGHT" type double,
"USERNAME" type string,
"JFSENDFLAG" type double,
"ISRECEIVED" type string,
"SPECIALMEMO" type string,
"TOPROCESSID" type string,
"JXLOCKED" type string
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_ZY_JG_PROCESS_parser
TYPE rcd
SCHEMA T_ZY_JG_PROCESS_schema;
CREATE TABLE T_ZY_JG_PROCESS using T_ZY_JG_PROCESS_parser;
create index T_ZY_JG_PROCESS_index on table T_ZY_JG_PROCESS(DEPTID);
create map PROCESSID_map on table T_ZY_JG_PROCESS
KEY (PROCESSID)
VALUE (1)
TYPE string
where OUTCARBILLTIME >= TO_DATE ('2012-07-02', '%Y-%m-%d');
create map DEPTID_map on table T_ZY_JG_PROCESS
KEY (DEPTID)
VALUE (1)
TYPE string
where OUTCARBILLTIME >= TO_DATE ('2012-07-02', '%Y-%m-%d');
create schema T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_schema
source type CSV
fields(
"DEPTID" type string,
"CHANGEOWNERID" type string,
"PREOWNERNO" type string,
"NEWOWNERNO" type string,
"PROOWNERNAME" type string,
"CHANGEDATE" type datetime,
"NEWOWNERNAME" type string,
"NETWEIGHT" type double,
"GROSSWEIGHT" type double,
"SHEETNUM" type double,
"GOODSID" type string,
"CHANGEOWNERNO" type string,
"DEPTID_1" type string
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_parser
TYPE rcd
SCHEMA T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_schema;
CREATE TABLE T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL using T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_parser;
create index T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_index on table T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL(DEPTID);
create schema union_all_schema
source type CSV
fields(
"GOODSID" type string,
"OWNERID" type string,
"OWNERNAME" type string,
"GRADENUMXH" type double,
"SINGLESHEETNUM" type double,
"NETWEIGHT" type double,
"GROSSWEIGHT" type double,
"SHEETNUM" type double
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER union_all_parser
TYPE rcd
SCHEMA union_all_schema;
CREATE TABLE union_all using union_all_parser;
create index union_all_index on table union_all(OWNERID);
create schema join_1_schema
source type CSV
fields(
"PREGOODSID" type string,
"OWNERID" type string,
"GRADENUMXH" type double,
"SINGLESHEETNUM" type double,
"SIDEMARK" type string,
"SHIPNAME" type string,
"NETWEIGHT" type double,
"OWNERNAME" type string,
"GROSSWEIGHT" type double,
"SHEETNUM" type double,
"DEPTID" type string,
"R_RECORD_IS_DELETED" type double,
"GOODSID" type string,
"GOODSID_1" type string
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER join_1_parser
TYPE rcd
SCHEMA join_1_schema;
CREATE TABLE join_1 using join_1_parser;
create index join_1_index on table join_1(OWNERID);
create statistics model join_1_model on table join_1
group by (PREGOODSID,OWNERID,SIDEMARK,SHIPNAME)
measures (sum(GRADENUMXH),sum(SINGLESHEETNUM),sum(NETWEIGHT),sum(GROSSWEIGHT),sum(SHEETNUM));
create schema join_2_schema
source type CSV
fields(
"OWNERID" type string,
"OWNERNAME" type string,
"GRADENUMXH" type double,
"SINGLESHEETNUM" type double,
"SIDEMARK" type string,
"SHIPNAME" type string,
"NETWEIGHT" type double,
"GROSSWEIGHT" type double,
"SHEETNUM" type double,
"PACKNUM" type string,
"MANAGENO" type string,
"GRADENUM" type string,
"PREGOODSID" type string,
"GOODSLOCID" type string,
"DEPTID" type string,
"R_RECORD_IS_DELETED" type double,
"GOODSID" type string,
"KINDNAME" type string,
"SHOPSIGNNAME" type string,
"PRODAREANAME" type string,
"FIRSTINDATE" type datetime
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER join_2_parser
TYPE rcd
SCHEMA join_2_schema;
CREATE TABLE join_2 using join_2_parser;
create index join_2_index on table join_2(OWNERID);
<file_sep>/schema/T_ZY_CK_OUTPACK.sql
use db;
create schema T_ZY_CK_OUTPACK_schema
source type CSV
fields(
"outpackid" type str,
"deptid" type str,
"outbillno" type str,
"goodsid" type str,
"packnum" type str,
"barcode" type str,
"goodslocid" type str,
"ownername" type str,
"ownerno" type str,
"goodsownermanagenum" type str,
"goodsownerpacknum" type str,
"managenum" type str,
"inbegintime" type datetime,
"contractno" type str,
"oldgoodsstatename" type str,
"oldgoodsstateno" type str,
"goodsstatename" type str,
"goodsstateno" type str,
"goodstypename" type str,
"goodstype" type str,
"kindname" type str,
"kindno" type str,
"shopsignname" type str,
"shopsignid" type str,
"prodareaname" type str,
"prodareaid" type str,
"goodsbatchnum" type str,
"gradenum" type str,
"gradenumthick" type double,
"gradenumwidth" type double,
"gradenumlength" type double,
"grossweight" type double,
"outgrossweight" type double,
"outnetweight" type double,
"netweight" type double,
"poundweight" type double,
"checkweight" type double,
"sheetnum" type double,
"packtype" type double,
"qualitymemo" type double,
"firstinownerid" type double,
"firstindatetime" type datetime,
"inbillid" type str,
"materialgoodsid" type str,
"intype" type str,
"memo" type str,
"custbill" type str,
"sendflag" type double,
"velflag" type double,
"r_record_create_date" type datatime,
"r_record_create_user"type str,
"r_record_update_date" type datetime,
"r_record_update_user" type str,
"r_record_is_deleted" type double,
"foreignlistno" type str,
"createid" type str,
"creatername" type str,
"sidemark" type str,
"stocktype" type str,
"outbillpickownername" type str,
"r_record_change_flag" type double,
"incusttime" type datetime,
"custday" type double,
"sdprice" type str,
"outtype" type str,
"extsyskindid" type str,
"extsysshopsignid" type str,
"extsysgradenum" type str,
"prodstoveno" type str,
"check_type" type str,
"singlesheetnum" type double,
"singlesheet" type double,
"gradenumxh" type double,
"outbillpickownerno" type str,
"outpassword" type str,
"shipno" type str,
"shipname" type str,
"shipcd" type str,
"shiplevel" type str,
"sort_emp" type str,
"length_product_max" type double,
"packtypename" type str,
"dbckflag" type double,
"dbowner" type str,
"dispersesheet" type double,
"outcarno" type str,
"outcusttime" type datetime,
"ply" type double,
"originoutsheetnum" type double,
"outsheetnum" type double,
"originoutnetweight" type double,
"issplit" type double,
"preforeignlistno"type str,
"drawno" type str,
"handno" type str,
"saveno" type str,
"loclv2" type str,
"gradenumwhdw" type str,
"optflag" type double,
"payedflag" type str,
"sale_price_at" type double,
"price" type double,
"changeownertimes" type double,
"contract_id" type str,
"contract_subid" type str,
"jyzxflag" type double,
"outbilldatetime" type datetime,
"rollply" type double,
"readyout" type datetime,
"validdatetime" type datetime,
"lockcount" type double default value "0",
"loclv1"type str
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "NULL";
CREATE PARSER T_ZY_CK_OUTPACK_parser
TYPE rcd
SCHEMA T_ZY_CK_OUTPACK_schema;
CREATE TABLE T_ZY_CK_OUTPACK using T_ZY_CK_OUTPACK_parser;
CREATE INDEX T_ZY_CK_OUTPACK_index ON TABLE T_ZY_CK_OUTPACK(price);<file_sep>/uncomplete/JINSHANG-78/jinshang-78.sql
SELECT
*
FROM
(
SELECT
tt10.*, '' ownername
FROM
(
(
SELECT
ownerid,
manageno,
SUM (qcnetweight) qcnetweight,
SUM (INnetweight) INnetweight,
SUM (JGRnetweight) JGRnetweight,
SUM (GHRnetweight) GHRnetweight,
SUM (OUTnetweight) OUTnetweight,
SUM (GHOnetweight) GHOnetweight,
SUM (JGCnetweight) JGCnetweight,
SUM (QMnetweight) QMnetweight,
SUM (js) js,
SUM (sheetnum) sheetnum,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
(
(
SELECT
ownerid,
manageno,
SUM (netweight) qcnetweight,
0 INnetweight,
0 JGRnetweight,
0 GHRnetweight,
0 OUTnetweight,
0 GHOnetweight,
0 JGCnetweight,
0 QMnetweight,
0 js,
0 sheetnum,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
(
SELECT
ownerid,
'' ownername,
manageno,
SUM (netweight) netweight,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
T_GG_SJ_Goods
WHERE
goodsstateno = '20'
AND deptid = '0301'
GROUP BY
ownerid,
manageno
UNION ALL
SELECT
ownerid,
'' ownername,
manageno ,- SUM (netweight) netweight,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
T_ZY_RK_InBillDetail
WHERE
deptid = '0301'
AND (goodsstateno = '20')
AND firstindate < TO_DATE ('2015/8/4', 'yyyy-mm-dd')
AND firstindate >= TO_DATE ('2015/7/1', 'yyyy-mm-dd')
GROUP BY
ownerid,
manageno
UNION ALL
SELECT
ownerid,
'' ownername,
manageno ,- SUM (netweight) netweight,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
T_ZY_JG_ProcArticleDtl
WHERE
deptid = '0301'
AND firstindate < TO_DATE ('2015/8/4', 'yyyy-mm-dd')
AND firstindate >= TO_DATE ('2015/7/1', 'yyyy-mm-dd')
AND goodsstateno = '130'
GROUP BY
ownerid,
manageno
UNION ALL
SELECT
newownerno aownerid,
newownername ownername,
managenum manageno ,- SUM (netweight) netweight,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
(
SELECT
A .newownerno,
A .newownername,
(
CASE
WHEN (
managenum IS NULL
OR managenum = ''
) THEN
premanagenum
ELSE
managenum
END
) managenum,
b.shopsignid,
b.shopsignname,
b.prodareaid,
b.prodareaname,
b.gradenum,
b.netweight,
b.kindname,
b.kindno
FROM
(
SELECT
deptid,
ChangeOwnerID,
newownerno,
'' newownername
FROM
T_ZY_GH_ChangeOwnerList
WHERE
DeptID = '0301'
AND ChangeDate < TO_DATE ('2015/8/4', 'yyyy-mm-dd')
AND changedate >= TO_DATE ('2015/7/1', 'yyyy-mm-dd')
) A
LEFT JOIN T_ZY_GH_ChangeOwnerDetail b ON A .changeownerid = b.changeownerno
AND A .deptid = b.deptid
) tb1
GROUP BY
newownerno,
newownername,
managenum
UNION ALL
SELECT
ownerno,
'' ownername,
managenum,
SUM (netweight) netweight,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
T_ZY_CK_OutPack
WHERE
deptid = '0301'
AND OutCustTime >= TO_DATE ('2015/7/1', 'yyyy-mm-dd')
AND OutCustTime < TO_DATE ('2015/8/4', 'yyyy-mm-dd')
GROUP BY
ownerno,
managenum
UNION ALL
SELECT
ownerid,
'' ownername,
manageno,
SUM (netweight) anetweight,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
T_ZY_JG_ProcMaterialDtl
WHERE
deptid = '0301'
AND EXISTS (
SELECT
1
FROM
T_ZY_JG_PROCESS
WHERE
ProcessID = T_ZY_JG_ProcMaterialDtl.ProcessID
AND deptid = T_ZY_JG_ProcMaterialDtl.deptid
AND OutCarBillTime >= TO_DATE ('2015/7/1', 'yyyy-mm-dd')
AND OutCarBillTime < TO_DATE ('2015/8/4', 'yyyy-mm-dd')
)
GROUP BY
ownerid,
manageno
UNION ALL
SELECT
preownerno,
proownername,
premanagenum,
SUM (netweight) anetweight,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
(
SELECT
A .preownerno,
A .proownername,
b.premanagenum,
b.netweight,
b.gradenum,
b.shopsignname,
b.shopsignid,
b.prodareaname,
b.prodareaid,
b.kindno,
b.kindname
FROM
(
SELECT
deptid,
ChangeOwnerID,
preownerno,
'' proownername
FROM
T_ZY_GH_ChangeOwnerList
WHERE
DeptID = '0301'
AND ChangeDate < TO_DATE ('2015/8/4', 'yyyy-mm-dd')
AND changedate >= TO_DATE ('2015/7/1', 'yyyy-mm-dd')
) A
LEFT JOIN T_ZY_GH_ChangeOwnerDetail b ON A .changeownerid = b.changeownerno
AND A .deptid = b.deptid
) tb1
GROUP BY
preownerno,
proownername,
premanagenum
) tbx
GROUP BY
ownerid,
ownername,
manageno
)
UNION ALL
(
SELECT
ownerid,
manageno,
0 qcnetweight,
SUM (netweight) INnetweight,
0 JGRnetweight,
0 GHRnetweight,
0 OUTnetweight,
0 GHOnetweight,
0 JGCnetweight,
0 QMnetweight,
0 js,
0 sheetnum,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
T_ZY_RK_InBillDetail
WHERE
deptid = '0301'
AND goodsstateno = '20'
AND firstindate < TO_DATE ('2015/8/1', 'yyyy-mm-dd')
AND firstindate >= TO_DATE ('2015/7/1', 'yyyy-mm-dd')
GROUP BY
ownerid,
manageno
)
UNION ALL
(
SELECT
ownerid,
manageno,
0 qcnetweight,
0 INnetweight,
SUM (netweight) JGRnetweight,
0 GHRnetweight,
0 OUTnetweight,
0 GHOnetweight,
0 JGCnetweight,
0 QMnetweight,
0 js,
0 sheetnum,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
T_ZY_JG_ProcArticleDtl
WHERE
deptid = '0301'
AND firstindate <= TO_DATE ('2015/8/1', 'yyyy-mm-dd')
AND firstindate >= TO_DATE ('2015/7/1', 'yyyy-mm-dd')
AND goodsstateno = '130'
GROUP BY
ownerid,
manageno
)
UNION ALL
(
SELECT
newownerno ownerid,
managenum manageno,
0 qcnetweight,
0 INnetweight,
0 JGRnetweight,
SUM (netweight) GHRnetweight,
0 OUTnetweight,
0 GHOnetweight,
0 JGCnetweight,
0 QMnetweight,
0 js,
0 sheetnum,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
(
SELECT
A .newownerno,
A .newownername,
(
CASE
WHEN (
managenum IS NULL
OR managenum = ''
) THEN
premanagenum
ELSE
managenum
END
) managenum,
b.shopsignid,
b.shopsignname,
b.prodareaid,
b.prodareaname,
b.gradenum,
b.netweight,
b.kindno,
b.kindname
FROM
(
SELECT
deptid,
ChangeOwnerID,
newownerno,
'' newownername
FROM
T_ZY_GH_ChangeOwnerList
WHERE
DeptID = '0301'
AND ChangeDate >= TO_DATE ('2015/7/1', 'yyyy-mm-dd')
AND changedate < TO_DATE ('2015/8/1', 'yyyy-mm-dd')
) A
LEFT JOIN T_ZY_GH_ChangeOwnerDetail b ON A .changeownerid = b.changeownerno
AND A .deptid = b.deptid
) tb1
GROUP BY
newownerno,
newownername,
managenum
)
UNION ALL
(
SELECT
ownerno ownerid,
managenum manageno,
0 qcnetweight,
0 INnetweight,
0 JGRnetweight,
0 GHRnetweight,
SUM (netweight) OUTnetweight,
0 GHOnetweight,
0 JGCnetweight,
0 QMnetweight,
0 js,
0 sheetnum,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
T_ZY_CK_OutPack
WHERE
deptid = '0301'
AND OutCustTime >= TO_DATE ('2015/7/1', 'yyyy-mm-dd')
AND OutCustTime < TO_DATE ('2015/8/1', 'yyyy-mm-dd')
GROUP BY
ownerno,
managenum
)
UNION ALL
(
SELECT
PREOWNERNO ownerid,
managenum manageno,
0 qcnetweight,
0 INnetweight,
0 JGRnetweight,
0 GHRnetweight,
0 OUTnetweight,
SUM (netweight) GHOnetweight,
0 JGCnetweight,
0 QMnetweight,
0 js,
0 sheetnum,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
(
SELECT
A .PREOWNERNO,
A .PRoOWNERName,
(
CASE
WHEN (
premanagenum IS NULL
OR premanagenum = ''
) THEN
managenum
ELSE
premanagenum
END
) managenum,
b.netweight,
b.gradenum,
b.shopsignname,
b.shopsignid,
b.kindname,
b.kindno,
b.prodareaname,
b.prodareaid
FROM
(
SELECT
deptid,
ChangeOwnerID,
PReOWNERNO,
'' PRoOWNERName
FROM
T_ZY_GH_ChangeOwnerList
WHERE
DeptID = '0301'
AND changedate < TO_DATE ('2015/8/1', 'yyyy-mm-dd')
AND changedate >= TO_DATE ('2015/7/1', 'yyyy-mm-dd')
) A
LEFT JOIN T_ZY_GH_ChangeOwnerDetail b ON A .changeownerid = b.changeownerno
AND A .deptid = b.deptid
) tb1
GROUP BY
PREOWNERNO,
PRoOWNERName,
managenum
)
UNION ALL
(
SELECT
ownerid,
manageno,
0 qcnetweight,
0 INnetweight,
0 JGRnetweight,
0 GHRnetweight,
0 OUTnetweight,
0 GHOnetweight,
SUM (netweight) JGCnetweight,
0 QMnetweight,
0 js,
0 sheetnum,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
T_ZY_JG_ProcMaterialDtl
WHERE
deptid = '0301'
AND EXISTS (
SELECT
1
FROM
T_ZY_JG_PROCESS
WHERE
ProcessID = T_ZY_JG_ProcMaterialDtl.ProcessID
AND deptid = T_ZY_JG_ProcMaterialDtl.deptid
AND OutCarBillTime >= TO_DATE ('2015/7/1', 'yyyy-mm-dd')
AND OutCarBillTime < TO_DATE ('2015/8/1', 'yyyy-mm-dd')
)
GROUP BY
ownerid,
manageno
)
UNION ALL
(
SELECT
ownerid,
manageno,
0 qcnetweight,
0 INnetweight,
0 JGRnetweight,
0 GHRnetweight,
0 OUTnetweight,
0 GHOnetweight,
0 JGCnetweight,
SUM (netweight) QMnetweight,
SUM (js) js,
SUM (sheetnum) sheetnum,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
(
SELECT
ownerid,
'' ownername,
manageno,
SUM (netweight) netweight,
COUNT js,
SUM (sheetnum) sheetnum,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
T_GG_SJ_Goods
WHERE
goodsstateno = '20'
AND deptid = '0301'
GROUP BY
ownerid,
manageno
UNION ALL
SELECT
ownerid,
'' ownername,
manageno ,- SUM (netweight) netweight ,- COUNT js ,- SUM (sheetnum) sheetnum,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
T_ZY_RK_InBillDetail
WHERE
deptid = '0301'
AND (goodsstateno = '20')
AND firstindate < TO_DATE ('2015/8/4', 'yyyy-mm-dd')
AND firstindate >= TO_DATE ('2015/8/1', 'yyyy-mm-dd')
GROUP BY
ownerid,
manageno
UNION ALL
SELECT
ownerid,
'' ownername,
manageno ,- SUM (netweight) netweight ,- COUNT js ,- SUM (sheetnum) sheetnum,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
T_ZY_JG_ProcArticleDtl
WHERE
deptid = '0301'
AND firstindate < TO_DATE ('2015/8/4', 'yyyy-mm-dd')
AND firstindate >= TO_DATE ('2015/8/1', 'yyyy-mm-dd')
AND goodsstateno = '130'
GROUP BY
ownerid,
manageno
UNION ALL
SELECT
newownerno ownerid,
newownername ownername,
managenum manageno ,- SUM (netweight) netweight,
- COUNT js ,- SUM (sheetnum) sheetnum,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
(
SELECT
A .newownerno,
A .newownername,
(
CASE
WHEN (
managenum IS NULL
OR managenum = ''
) THEN
premanagenum
ELSE
managenum
END
) managenum,
b.netweight,
b.sheetnum,
b.gradenum,
b.shopsignname,
b.shopsignid,
b.kindname,
b.kindno,
b.prodareaname,
b.prodareaid
FROM
(
SELECT
deptid,
ChangeOwnerID,
newownerno,
'' newownername
FROM
T_ZY_GH_ChangeOwnerList
WHERE
DeptID = '0301'
AND ChangeDate < TO_DATE ('2015/8/4', 'yyyy-mm-dd')
AND changedate >= TO_DATE ('2015/8/1', 'yyyy-mm-dd')
) A
LEFT JOIN T_ZY_GH_ChangeOwnerDetail b ON A .changeownerid = b.changeownerno
AND A .deptid = b.deptid
) tb1
GROUP BY
newownerno,
newownername,
managenum
UNION ALL
SELECT
ownerno,
'' ownername,
managenum,
SUM (netweight) netweight,
COUNT js,
SUM (sheetnum) sheetnum,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
T_ZY_CK_OutPack
WHERE
deptid = '0301'
AND OutCustTime >= TO_DATE ('2015/8/1', 'yyyy-mm-dd')
AND OutCustTime < TO_DATE ('2015/8/4', 'yyyy-mm-dd')
GROUP BY
ownerno,
managenum
UNION ALL
SELECT
ownerid,
'' ownername,
manageno,
SUM (netweight) netweight,
COUNT js,
SUM (sheetnum) sheetnum,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
T_ZY_JG_ProcMaterialDtl
WHERE
deptid = '0301'
AND EXISTS (
SELECT
1
FROM
T_ZY_JG_PROCESS
WHERE
ProcessID = T_ZY_JG_ProcMaterialDtl.ProcessID
AND deptid = T_ZY_JG_ProcMaterialDtl.deptid
AND OutCarBillTime >= TO_DATE ('2015/8/1', 'yyyy-mm-dd')
AND OutCarBillTime < TO_DATE ('2015/8/4', 'yyyy-mm-dd')
)
GROUP BY
ownerid,
manageno
UNION ALL
SELECT
preownerno,
proownername,
premanagenum,
SUM (netweight) netweight,
COUNT js,
SUM (sheetnum) sheetnum,
MAX (kindno) kindno,
MAX (kindname) kindname,
MAX (prodareaid) prodareaid,
MAX (prodareaname) prodareaname,
MAX (shopsignid) shopsignid,
MAX (shopsignname) shopsignname,
MAX (gradenum) gradenum
FROM
(
SELECT
A .preownerno,
A .proownername,
b.premanagenum,
b.netweight,
b.sheetnum,
b.gradenum,
b.shopsignname,
b.shopsignid,
b.kindno,
b.kindname,
b.prodareaname,
b.prodareaid
FROM
(
SELECT
deptid,
ChangeOwnerID,
preownerno,
'' proownername
FROM
T_ZY_GH_ChangeOwnerList
WHERE
DeptID = '0301'
AND ChangeDate < TO_DATE ('2015/8/4', 'yyyy-mm-dd')
AND changedate >= TO_DATE ('2015/8/1', 'yyyy-mm-dd')
) A
LEFT JOIN T_ZY_GH_ChangeOwnerDetail b ON A .changeownerid = b.changeownerno
AND A .deptid = b.deptid
) tb1
GROUP BY
preownerno,
proownername,
premanagenum
) tbx
GROUP BY
ownerid,
ownername,
manageno
)
)
GROUP BY
ownerid,
manageno
) tt10
)
) tt30
WHERE
ownerid IS NOT NULL
AND NOT (
qcnetweight = 0
AND INnetweight = 0
AND JGRnetweight = 0
AND GHRnetweight = 0
AND OUTnetweight = 0
AND GHOnetweight = 0
AND JGCnetweight = 0
AND QMnetweight = 0
AND js = 0
AND sheetnum = 0
)
AND ownerid = 'KH00001'
AND manageno = 'M'
ORDER BY
ownerid,
manageno;
<file_sep>/uncomplete/JINSHANG-72/jinshang-72.sql
SELECT *
FROM
(SELECT rownum AS rownum_,
aa.*
FROM
(SELECT /*+ index(g,T_GG_SJ_GOODS_INDX02) */ nvl(DEPTID, 'null') AS DEPTID,
(SELECT deptname
FROM wmsdba.t_common_dept d
WHERE d.deptid = g.deptid) AS DEPTNAME,
nvl(GRADENUM, 'null') AS GRADENUM,
nvl(SHOPSIGNID, 'null') AS SHOPSIGNID,
nvl(SHOPSIGNNAME, 'null') AS SHOPSIGNNAME,
nvl(PRODAREAID, 'null') AS PRODAREAID,
nvl(PRODAREANAME, 'null') AS PRODAREANAME,
nvl(KINDNO, 'null') AS KINDNO,
nvl(KINDNAME, 'null') AS KINDNAME,
nvl(ownername, 'null') AS OWNERSHORT,
nvl(GOODSOWNERPACKNO, 'null') AS GOODSOWNERPACKNO,
nvl(SUM(NETWEIGHT), 0) AS NETWEIGHT,
nvl(SUM(GROSSWEIGHT), 0) AS GROSSWEIGHT,
nvl(SUM(SHEETNUM), 0) AS SHEETNUM
FROM wmsdba.t_gg_sj_goods g,
ST.T_XTSZ_BIND b
WHERE 1 = 1
AND g.deptid = b.dept_id
AND g.ownerid = b.owner_id
AND b.alive_flag = '1'
AND b.seg_no = '080001'
AND goodsstateno = '20'
AND KINDNAME LIKE '%' ||'²»Ðâ¸Ö'|| '%'
AND SHOPSIGNNAME LIKE '%'|| 'spcd'|| '%'
AND GRADENUM LIKE '%' ||'11*11*11'|| '%'
AND PRODAREANAME LIKE '%'||'°ÍÎ÷'|| '%'
AND GOODSOWNERPACKNO LIKE '%' ||'123'|| '%'
AND FIRSTINDATE >= to_date('2015-07-16', 'yyyy-MM-dd')
AND FIRSTINDATE < to_date('2015-07-16', 'yyyy-MM-dd') + 1
GROUP BY g.deptid,
GRADENUM,
SHOPSIGNID,
SHOPSIGNNAME,
PRODAREAID,
PRODAREANAME,
KINDNO,
KINDNAME,
ownername,
GOODSOWNERPACKNO) aa)
WHERE rownum_ > 0
AND rownum_ <= 10<file_sep>/uncomplete/JINSHANG-100/2_init.sql
create job 1_job(1)
begin
dataset file T_ZY_CK_CLEAR_1_dataset
(
schema: "T_ZY_CK_CLEAR_schema",
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_CK_CLEAR.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc index T_ZY_CK_CLEAR_index
(
inputs:T_ZY_CK_CLEAR_1_dataset,
table:T_ZY_CK_CLEAR
);
dataproc doc T_ZY_CK_CLEAR_doc
(
inputs:T_ZY_CK_CLEAR_1_dataset,
table:T_ZY_CK_CLEAR
);
dataproc map T_ZY_CK_CLEAR_1_map
(
inputs:T_ZY_CK_CLEAR_1_dataset,
table:T_ZY_CK_CLEAR
);
end;
run job 1_job (threads:8);
create job 2_job(1)
begin
dataset file T_ZY_JG_PROCESS_1_dataset
(
schema: T_ZY_JG_PROCESS_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_JG_PROCESS.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc index P_index
(
inputs:T_ZY_JG_PROCESS_1_dataset,
table:T_ZY_JG_PROCESS
);
dataproc doc P_doc
(
inputs:T_ZY_JG_PROCESS_1_dataset,
table:T_ZY_JG_PROCESS
);
dataproc map P_1_map
(
inputs:T_ZY_JG_PROCESS_1_dataset,
table:T_ZY_JG_PROCESS
);
end;
run job 2_job (threads:8);
create job 3_job(3)
begin
dataset file T_GG_SJ_GOODS_dataset
(
schema: "T_GG_SJ_GOODS_schema",
filename: "/home/zhaoshun/Data/jinshang_New/T_GG_SJ_GOODS.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataset file T_ZY_RK_INBILLDETAIL_dataset
(
schema: T_ZY_RK_INBILLDETAIL_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_RK_INBILLDETAIL.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataset file T_ZY_JG_PROCARTICLEDTL_dataset
(
schema: T_ZY_JG_PROCARTICLEDTL_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_JG_PROCARTICLEDTL.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataset file T_ZY_GH_CHANGEOWNERLIST_dataset
(
schema: T_ZY_GH_CHANGEOWNERLIST_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_GH_CHANGEOWNERLIST.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataset file T_ZY_GH_CHANGEOWNERDETAIL_dataset
(
schema: T_ZY_GH_CHANGEOWNERDETAIL_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_GH_CHANGEOWNERDETAIL.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataset file T_ZY_CK_OUTPACK_dataset
(
schema: T_ZY_CK_OUTPACK_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_CK_OUTPACK.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataset file T_ZY_JG_PROCMATERIALDTL_dataset
(
schema: T_ZY_JG_PROCMATERIALDTL_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_JG_PROCMATERIALDTL.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataset file T_ZY_CK_CLEAR_dataset
(
schema: T_ZY_CK_CLEAR_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_CK_CLEAR.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataset file T_ZY_JG_PROCESS_dataset
(
schema: T_ZY_JG_PROCESS_schema,
filename: "/home/zhaoshun/Data/jinshang_New/T_ZY_JG_PROCESS.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc select T_ZY_GH_CHANGEOWNERLIST_select
(
fields:
(
(fname:"DEPTID"),
(fname:"CHANGEOWNERID"),
(fname:"NEWOWNERNO"),
(fname:"NEWOWNERNAME"),
(fname:"PREOWNERNO"),
(fname:"PROOWNERNAME"),
(fname:"CHANGEDATE")
),
inputs: "T_ZY_GH_CHANGEOWNERLIST_dataset",
order_by:("DEPTID","CHANGEOWNERID"),
conditions:"DEPTID = '101'
AND CHANGEDATE >= TO_DATE ('2012-07-02', '%Y-%m-%d')"
);
dataproc select T_ZY_GH_CHANGEOWNERDETAIL_select
(
fields:
(
(fname:"GOODSID"),
(fname:"NETWEIGHT"),
(fname:"GROSSWEIGHT"),
(fname:"SHEETNUM"),
(fname:"CHANGEOWNERNO"),
(fname:"DEPTID",alias:"DEPTID_1",type:"string")
),
inputs: "T_ZY_GH_CHANGEOWNERDETAIL_dataset",
order_by:("DEPTID_1","CHANGEOWNERNO")
);
dataproc join T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_join
(
inputs:
(left_input:T_ZY_GH_CHANGEOWNERLIST_select,right_input:T_ZY_GH_CHANGEOWNERDETAIL_select),
join_keys:
(
("left_input.DEPTID","right_input.DEPTID_1"),
("left_input.CHANGEOWNERID","right_input.CHANGEOWNERNO")
)
);
dataproc index T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_index
(
inputs:T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_join,
table:T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL
);
dataproc doc T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_doc
(
inputs:T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_join,
table:T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL,
format:T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_parser,
fields:("DEPTID","CHANGEOWNERID","PREOWNERNO","NEWOWNERNO","PROOWNERNAME","CHANGEDATE","NEWOWNERNAME","NETWEIGHT","GROSSWEIGHT","SHEETNUM","GOODSID","CHANGEOWNERNO","DEPTID_1")
);
dataproc select T_GG_SJ_GOODS_select
(
fields:
(
(fname:"GOODSID"),
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"GRADENUMXH"),
(fname:"SINGLESHEETNUM"),
(fname:"NETWEIGHT"),
(fname:"GROSSWEIGHT"),
(fname:"SHEETNUM")
),
inputs: "T_GG_SJ_GOODS_dataset",
conditions:"GOODSSTATENO = '110'
AND R_RECORD_IS_DELETED = 0
AND DEPTID = '101'",
order_by:("GOODSID")
);
dataproc select T_ZY_RK_INBILLDETAIL_select
(
fields:
(
(fname:"GOODSID"),
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"GRADENUMXH"),
(fname:"SINGLESHEETNUM"),
(fname:"NETWEIGHT"),
(fname:"GROSSWEIGHT"),
(fname:"SHEETNUM")
),
inputs: "T_ZY_RK_INBILLDETAIL_dataset",
conditions:"DEPTID = '101'
AND GOODSSTATENO = '110'
AND FIRSTINDATE >= TO_DATE ('2012-07-02', '%Y-%m-%d')",
order_by:("GOODSID")
);
dataproc select T_ZY_JG_PROCARTICLEDTL_select
(
fields:
(
(fname:"PROCARTICLEDTLID"),
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"\'0\'",alias:"GRADENUMXH",type:"double"),
(fname:"\'0\'",alias:"SINGLESHEETNUM",type:"double"),
(fname:"NETWEIGHT"),
(fname:"GROSSWEIGHT"),
(fname:"SHEETNUM")
),
inputs: "T_ZY_JG_PROCARTICLEDTL_dataset",
conditions:"DEPTID = '101'
AND FIRSTINDATE >= TO_DATE ('2012-07-02', '%Y-%m-%d')
AND GOODSSTATENO = '130'",
order_by:("OWNERID")
);
dataproc select T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_select
(
fields:
(
(fname:"GOODSID"),
(fname:"NEWOWNERNO",alias:"OWNERID",type:"string"),
(fname:"NEWOWNERNAME",alias:"OWNERNAME",type:"string"),
(fname:"\'0\'",alias:"GRADENUMXH",type:"double"),
(fname:"\'0\'",alias:"SINGLESHEETNUM",type:"double"),
(fname:"NETWEIGHT"),
(fname:"GROSSWEIGHT"),
(fname:"SHEETNUM")
),
inputs: "T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_join",
order_by:("GOODSID")
);
dataproc select T_ZY_CK_OUTPACK_select
(
fields:
(
(fname:"GOODSID"),
(fname:"OWNERNO"),
(fname:"OWNERNAME"),
(fname:"GRADENUMXH"),
(fname:"SINGLESHEETNUM"),
(fname:"NETWEIGHT"),
(fname:"GROSSWEIGHT"),
(fname:"SHEETNUM")
),
inputs: "T_ZY_CK_OUTPACK_dataset",
conditions:"DEPTID = '101'
AND OUTCUSTTIME >= TO_DATE ('2012-07-02', '%Y-%m-%d')",
order_by:("GOODSID")
);
dataproc select T_GG_SJ_GOODS_2_select
(
fields:
(
(fname:"GOODSID"),
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"GRADENUMXH"),
(fname:"SINGLESHEETNUM"),
(fname:"NETWEIGHT"),
(fname:"GROSSWEIGHT"),
(fname:"SHEETNUM")
),
inputs: "T_GG_SJ_GOODS_dataset",
conditions:"CREATDATE >= TO_DATE ('2012-07-02', '%Y-%m-%d')",
order_by:("GOODSID")
);
dataproc select T_ZY_JG_PROCMATERIALDTL_select
(
fields:
(
(fname:"GOODSID"),
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"\'0\'",alias:"GRADENUMXH",type:"double"),
(fname:"\'0\'",alias:"SINGLESHEETNUM",type:"double"),
(fname:"NETWEIGHT"),
(fname:"GROSSWEIGHT"),
(fname:"SHEETNUM")
),
inputs: "T_ZY_JG_PROCMATERIALDTL_dataset",
conditions:"vf_PROCESSID = 1
and vf_DEPTID = 1 ",
order_by:("GOODSID")
);
dataproc select T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_1_select
(
fields:
(
(fname:"GOODSID"),
(fname:"PREOWNERNO",alias:"OWNERID",type:"string"),
(fname:"PROOWNERNAME",alias:"OWNERNAME",type:"string"),
(fname:"\'0\'",alias:"GRADENUMXH",type:"double"),
(fname:"\'0\'",alias:"SINGLESHEETNUM",type:"double"),
(fname:"NETWEIGHT"),
(fname:"GROSSWEIGHT"),
(fname:"SHEETNUM")
),
inputs: "T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_join",
order_by:("GOODSID")
);
dataproc union 1_union
(
inputs:("T_GG_SJ_GOODS_select","T_ZY_RK_INBILLDETAIL_select","T_ZY_JG_PROCARTICLEDTL_select","T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_select","T_ZY_CK_OUTPACK_select","T_GG_SJ_GOODS_2_select","T_ZY_JG_PROCMATERIALDTL_select","T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_1_select")
);
dataproc select T_GG_SJ_GOODS_3_select
(
fields:
(
(fname:"PREGOODSID"),
(fname:"SIDEMARK"),
(fname:"SHIPNAME"),
(fname:"R_RECORD_IS_DELETED"),
(fname:"DEPTID"),
(fname:"GOODSID",alias:"GOODSID_1",type:"string")
),
inputs:"T_GG_SJ_GOODS_dataset",
conditions:"DEPTID = '101'
and R_RECORD_IS_DELETED = 0 ",
order_by:("GOODSID_1")
);
dataproc select 1_union_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"GRADENUMXH"),
(fname:"SINGLESHEETNUM"),
(fname:"NETWEIGHT"),
(fname:"GROSSWEIGHT"),
(fname:"SHEETNUM"),
(fname:"GOODSID")
),
inputs:"1_union",
order_by:("GOODSID")
);
dataproc leftjoin T_GG_SJ_GOODS_3_1_union_join
(
inputs:
(left_input:T_GG_SJ_GOODS_3_select,right_input:1_union_select),
join_keys:
(
("left_input.GOODSID_1","right_input.GOODSID")
)
);
dataproc select leftjoin_select
(
fields:
(
(fname:"PREGOODSID"),
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"sum(GRADENUMXH)",alias:"GRADENUMXH",type:"double"),
(fname:"sum(SINGLESHEETNUM)",alias:"SINGLESHEETNUM",type:"double"),
(fname:"SIDEMARK",alias:"SIDEMARK",type:"string"),
(fname:"SHIPNAME",alias:"SHIPNAME",type:"string"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_GG_SJ_GOODS_3_1_union_join",
group_by:(PREGOODSID,OWNERID,SIDEMARK,SHIPNAME)
);
dataproc select leftjoin_1_select
(
fields:
(
(fname:"PREGOODSID"),
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"sum(GRADENUMXH)",alias:"GRADENUMXH",type:"double"),
(fname:"sum(SINGLESHEETNUM)",alias:"SINGLESHEETNUM",type:"double"),
(fname:"SIDEMARK",alias:"SIDEMARK",type:"string"),
(fname:"SHIPNAME",alias:"SHIPNAME",type:"string"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_GG_SJ_GOODS_3_1_union_join",
group_by:(PREGOODSID,OWNERID,SIDEMARK,SHIPNAME)
);
dataproc statistics union_all_statistics
(
inputs:leftjoin_select,
table:join_1
);
dataproc select T_GG_SJ_GOODS_4_select
(
fields:
(
(fname:"GOODSLOCID"),
(fname:"DEPTID"),
(fname:"R_RECORD_IS_DELETED"),
(fname:"GOODSID",type:"string"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"MANAGENO")
),
inputs:"T_GG_SJ_GOODS_dataset",
conditions:"DEPTID = '101'
and R_RECORD_IS_DELETED = 0 ",
order_by:("GOODSID")
);
dataproc select leftjoin_2_select
(
fields:
(
(fname:"OWNERID"),
(fname:"\'0\'",alias:"OWNERNAME",type:"string"),
(fname:"GRADENUMXH"),
(fname:"SINGLESHEETNUM"),
(fname:"SIDEMARK"),
(fname:"SHIPNAME"),
(fname:"NETWEIGHT"),
(fname:"GROSSWEIGHT"),
(fname:"SHEETNUM"),
(fname:"PREGOODSID")
),
inputs:"leftjoin_1_select",
order_by:("PREGOODSID")
);
dataproc leftjoin T_GG_SJ_GOODS_4_join
(
inputs:
(left_input:T_GG_SJ_GOODS_4_select,right_input:leftjoin_2_select),
join_keys:
(
("left_input.GOODSID","right_input.PREGOODSID")
)
);
dataproc index join_2_1_index
(
inputs:T_GG_SJ_GOODS_4_join,
table:join_2
);
dataproc doc join_2_doc
(
inputs:T_GG_SJ_GOODS_4_join,
table:join_2,
format:join_2_parser,
fields:("OWNERID","OWNERNAME","GRADENUMXH","SINGLESHEETNUM","SIDEMARK","SHIPNAME","NETWEIGHT","GROSSWEIGHT","SHEETNUM","PACKNUM","MANAGENO","GRADENUM","PREGOODSID","GOODSLOCID","DEPTID","R_RECORD_IS_DELETED","GOODSID","KINDNAME","SHOPSIGNNAME","PRODAREANAME","FIRSTINDATE")
);
end;
run job 3_job (threads:8);
<file_sep>/uncomplete/JINSHANG-97/schema/T_ZY_CK_OUTBILL.sql
CREATE SCHEMA t_zy_ck_outbill_schema
SOURCE TYPE CSV
FIELDS(
"outbillid" type str,
"deptid" type str,
"foreignlistno" type str,
"outbillownername" type str,
"outbillownerno" type str,
"outbillpickownername" type str,
"outbillpickownerno" type str,
"outtype" type str,
"outcusttime" type datetime format '%Y/%m/%d %H:%M:%S',
"outbillempno" type str,
"outbillempname" type str,
"outbilltime" type datetime format '%Y/%m/%d %H:%M:%S',
"newmanagenum" type str,
"truckgroupno" type str,
"outcarno" type str,
"outbillmemo" type str,
"planno" type str,
"netweightsum" type double,
"sheetnumsum" type double,
"r_record_create_date" type datetime format '%Y/%m/%d %H:%M:%S',
"r_record_create_user" type str,
"r_record_update_date" type datetime format '%Y/%m/%d %H:%M:%S',
"r_record_update_user" type str,
"r_record_is_deleted" type double,
"outstate" type str,
"invoicekindid" type str,
"invoicekindname" type str,
"r_record_change_flag" type double,
"printcount" type double,
"maxprintcount" type double,
"issplit" type double,
"outtypename" type str,
"check_type" type str,
"operator" type str,
"outcardrivername" type str,
"idcode" type str,
"settlenum" type str,
"outpassword" type str,
"sendmessage" type str,
"dtlcount" type double,
"confirm_flag" type str default value '0',
"confirm_date" type datetime format '%Y/%m/%d %H:%M:%S',
"confirm_emp" type str,
"issettled" type double,
"deptto" type str,
"trans_type" type str,
"jfsendflag" type double,
"jyzxflag" type double,
"username" type str,
"billlock" type str
)
SCHEMA DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER t_zy_ck_outbill_parser
TYPE rcd
SCHEMA t_zy_ck_outbill_schema;
<file_sep>/complete/JINSHANG-110/add_1.sql
create job add_1_job(2)
begin
dataset file T_GG_SJ_GOODS_dataset_11
(
schema: T_GG_SJ_GOODS_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_gg_sj_goods/insert/insert.csv",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc select union_select
(
inputs: "T_GG_SJ_GOODS_dataset_11",
fields:
(
(fname:"netweight",alias:"store_amount",type:"double"),
(fname:"0",alias:"store_in_amount",type:"double"),
(fname:"0",alias:"store_out_amount",type:"double"),
(fname:"0",alias:"store_process_amount",type:"double")
),
distinct: true,
cache:true,
conditions:" goodsstateno = '110'
AND deptid NOT LIKE '03%'
AND deptid NOT LIKE 'ZZ%'
AND deptid NOT LIKE 'MG%' "
);
dataproc statistics T_GG_SJ_GOOS_statistics
(
inputs:"union_select",
table:union_all
);
end;
run job add_1_job (threads:8);
<file_sep>/complete/JINSHANG-102/2_init.sql
create job job0(1)
begin
dataset file T_ZY_CK_CLEAR_1_dataset
(
schema: "T_ZY_CK_CLEAR_schema",
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_CK_CLEAR.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_CK_CLEAR_syncher
(
inputs: T_ZY_CK_CLEAR_1_dataset,
database:db,
operator:add,
table:T_ZY_CK_CLEAR
);
end;
run job job0(threads:8);
create job 1_job(1)
begin
dataset file T_ZY_JG_PROCESS_1_dataset
(
schema: T_ZY_JG_PROCESS_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_JG_PROCESS.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_JG_PROCESS_syncher
(
inputs: T_ZY_JG_PROCESS_1_dataset,
database:db,
operator:add,
table:T_ZY_JG_PROCESS
);
end;
run job 1_job (threads:8);
create service service_1(3)
begin
dataset file T_GG_SJ_GOODS_dataset
(
schema: "T_GG_SJ_GOODS_schema",
filename: "/home/natt/Data/jinshang_Data_New/T_GG_SJ_GOODS.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataset file T_ZY_RK_INBILLDETAIL_dataset
(
schema: T_ZY_RK_INBILLDETAIL_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_RK_INBILLDETAIL.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataset file T_ZY_JG_PROCARTICLEDTL_dataset
(
schema: T_ZY_JG_PROCARTICLEDTL_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_JG_PROCARTICLEDTL.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataset file T_ZY_GH_CHANGEOWNERLIST_dataset
(
schema: T_ZY_GH_CHANGEOWNERLIST_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_GH_CHANGEOWNERLIST.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataset file T_ZY_GH_CHANGEOWNERDETAIL_dataset
(
schema: T_ZY_GH_CHANGEOWNERDETAIL_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_GH_CHANGEOWNERDETAIL.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataset file T_ZY_CK_OUTPACK_dataset
(
schema: T_ZY_CK_OUTPACK_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_CK_OUTPACK.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataset file T_ZY_JG_PROCMATERIALDTL_dataset
(
schema: T_ZY_JG_PROCMATERIALDTL_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_JG_PROCMATERIALDTL.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc select T_ZY_GH_CHANGEOWNERLIST_select
(
fields:
(
(fname:"DEPTID"),
(fname:"CHANGEOWNERID"),
(fname:"NEWOWNERNO"),
(fname:"NEWOWNERNAME"),
(fname:"PREOWNERNO"),
(fname:"PROOWNERNAME"),
(fname:"CHANGEDATE")
),
inputs: "T_ZY_GH_CHANGEOWNERLIST_dataset",
order_by:("DEPTID","CHANGEOWNERID"),
conditions:"DEPTID = '101'
AND CHANGEDATE >= TO_DATE ('2012-07-02', '%Y-%m-%d')",
cache : "true",
into: "T_ZY_GH_CHANGEOWNERLIST"
);
dataproc select T_ZY_GH_CHANGEOWNERDETAIL_select
(
fields:
(
(fname:"PREMANAGENUM"),
(fname:"CASE WHEN ( MANAGENUM IS NULL OR MANAGENUM = '') THEN PREMANAGENUM ELSE MANAGENUM END ",alias:"MANAGENUM",type:"string"),
(fname:"SHOPSIGNID"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREAID"),
(fname:"PRODAREANAME"),
(fname:"GRADENUM"),
(fname:"NETWEIGHT"),
(fname:"GROSSWEIGHT"),
(fname:"SHEETNUM"),
(fname:"FIRSTINDATE"),
(fname:"KINDNAME"),
(fname:"GOODSLOCID"),
(fname:"PACKNUM"),
(fname:"CHANGEOWNERNO"),
(fname:"DEPTID",alias:"DEPTID_1",type:"string"),
(fname:"GRADENUMXH"),
(fname:"SINGLESHEETNUM"),
(fname:"OWNERNAME"),
(fname:"GOODSOWNERMANAGENUM",alias:"GOODSOWNERMANAGENO",type:"string")
),
inputs: "T_ZY_GH_CHANGEOWNERDETAIL_dataset",
order_by:("DEPTID_1","CHANGEOWNERNO"),
cache : "true",
into: "T_ZY_GH_CHANGEOWNERDETAIL"
);
dataproc join T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_join
(
inputs:
(left_input:T_ZY_GH_CHANGEOWNERLIST_select,right_input:T_ZY_GH_CHANGEOWNERDETAIL_select),
join_keys:
(
("left_input.DEPTID","right_input.DEPTID_1"),
("left_input.CHANGEOWNERID","right_input.CHANGEOWNERNO")
),
into: "T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL"
);
dataproc select T_GG_SJ_GOODS_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"sum(GRADENUMXH)",alias:"GRADENUMXH",type:"double"),
(fname:"sum(SINGLESHEETNUM)",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_GG_SJ_GOODS_dataset",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE),
conditions:"GOODSSTATENO = '110'
AND R_RECORD_IS_DELETED = 0
AND DEPTID = '101'",
cache : "true",
into: "T_GG_SJ_GOODS"
);
dataproc select A_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"sum(GRADENUMXH)",alias:"GRADENUMXH",type:"double"),
(fname:"sum(SINGLESHEETNUM)",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_ZY_RK_INBILLDETAIL_dataset",
conditions:"DEPTID = '101'
AND GOODSSTATENO = '20'
AND FIRSTINDATE >= TO_DATE ('2012-07-02', '%Y-%m-%d')",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE),
into: "T_ZY_RK_INBILLDETAIL"
);
dataproc select T_ZY_RK_INBILLDETAIL_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"0-GRADENUMXH",alias:"GRADENUMXH",type:"double"),
(fname:"0-SINGLESHEETNUM",alias:"SINGLESHEETNUM",type:"double"),
(fname:"0-NETWEIGHT",alias:"NETWEIGHT",type:"double"),
(fname:"0-GROSSWEIGHT",alias:"GROSSWEIGHT",type:"double"),
(fname:"0-SHEETNUM",alias:"SHEETNUM",type:"double")
),
inputs: "A_select",
cache : "true",
into: "T_ZY_RK_INBILLDETAIL"
);
dataproc select B_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"0",alias:"GRADENUMXH",type:"double"),
(fname:"0",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_ZY_JG_PROCARTICLEDTL_dataset",
conditions:"DEPTID = '101'
AND FIRSTINDATE >= TO_DATE ('2012-07-02', '%Y-%m-%d')
AND GOODSSTATENO = '130'",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE),
into: "T_ZY_JG_PROCARTICLEDTL"
);
dataproc select T_ZY_JG_PROCARTICLEDTL_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"0",alias:"GRADENUMXH",type:"double"),
(fname:"0",alias:"SINGLESHEETNUM",type:"double"),
(fname:"0-NETWEIGHT",alias:"NETWEIGHT",type:"double"),
(fname:"0-GROSSWEIGHT",alias:"GROSSWEIGHT",type:"double"),
(fname:"0-SHEETNUM",alias:"SHEETNUM",type:"double")
),
inputs: "B_select",
cache : "true",
into: "T_ZY_JG_PROCARTICLEDTL"
);
dataproc select C_select
(
fields:
(
(fname:"NEWOWNERNO",alias:"OWNERID"),
(fname:"NEWOWNERNAME",alias:"OWNERNAME"),
(fname:"MANAGENUM",alias:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"0",alias:"GRADENUMXH",type:"double"),
(fname:"0",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_join",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE),
into: "T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL"
);
dataproc select T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_select
(
fields:
(
(fname:"NEWOWNERNO",alias:"OWNERID"),
(fname:"NEWOWNERNAME",alias:"OWNERNAME"),
(fname:"MANAGENUM",alias:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"0",alias:"GRADENUMXH",type:"double"),
(fname:"0",alias:"SINGLESHEETNUM",type:"double"),
(fname:"0-NETWEIGHT",alias:"NETWEIGHT",type:"double"),
(fname:"0-GROSSWEIGHT",alias:"GROSSWEIGHT",type:"double"),
(fname:"0-SHEETNUM",alias:"SHEETNUM",type:"double")
),
inputs: "C_select",
cache : "true",
into: "T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL"
);
dataproc select T_ZY_CK_OUTPACK_select
(
fields:
(
(fname:"OWNERNO"),
(fname:"OWNERNAME"),
(fname:"MANAGENUM",alias:"MANAGENO"),
(fname:"GOODSOWNERMANAGENUM",alias:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"sum(GRADENUMXH)",alias:"GRADENUMXH",type:"double"),
(fname:"sum(SINGLESHEETNUM)",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_ZY_CK_OUTPACK_dataset",
conditions:"DEPTID = '101'
AND OUTCUSTTIME >= TO_DATE ('2012-07-02', '%Y-%m-%d')",
group_by:(OWNERNO,OWNERNAME,MANAGENUM,GOODSOWNERMANAGENUM,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE),
cache : "true",
into: "T_ZY_CK_OUTPACK"
);
dataproc select T_GG_SJ_GOODS_2_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PACKNUM"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"sum(GRADENUMXH)",alias:"GRADENUMXH",type:"double"),
(fname:"sum(SINGLESHEETNUM)",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_GG_SJ_GOODS_dataset",
conditions:"CREATDATE >= TO_DATE ('2012-07-02', '%Y-%m-%d')",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE),
cache : "true",
into: "T_GG_SJ_GOODS"
);
dataproc select T_ZY_JG_PROCMATERIALDTL_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"0",alias:"GRADENUMXH",type:"double"),
(fname:"0",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_ZY_JG_PROCMATERIALDTL_dataset",
conditions:"vf_PROCESSID = '1'
and DEPTID = '101' ",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE),
cache : "true",
into: "T_ZY_JG_PROCMATERIALDTL"
);
dataproc select T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_1_select
(
fields:
(
(fname:"PREOWNERNO",alias:"OWNERID"),
(fname:"PROOWNERNAME",alias:"OWNERNAME"),
(fname:"PREMANAGENUM",alias:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"0",alias:"GRADENUMXH",type:"double"),
(fname:"0",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_join",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE),
cache : "true",
into: "T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL"
);
dataproc union 1_union
(
inputs:("T_GG_SJ_GOODS_select","T_ZY_RK_INBILLDETAIL_select","T_ZY_JG_PROCARTICLEDTL_select","T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_select","T_ZY_CK_OUTPACK_select","T_GG_SJ_GOODS_2_select","T_ZY_JG_PROCMATERIALDTL_select","T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_1_select"),
into:"union_all"
);
dataproc select union_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"sum(GRADENUMXH)",alias:"GRADENUMXH",type:"double"),
(fname:"sum(SINGLESHEETNUM)",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "1_union",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE),
cache : "true",
into: "union_all"
);
dataproc statistics union_all_statistics
(
inputs:union_select,
table:union_all
);
end;
start service service_1 {
"running_name" : "idx_job",
"interval" : 0,
"volume" : 1000000000,
"threads" : 4
};
create service service_2(3)
begin
dataset file T_GG_SJ_GOODS_del_dataset
(
schema: "T_GG_SJ_GOODS_schema",
filename: "/home/natt/Data/jinshang_Data_New/T_GG_SJ_GOODS.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataset file T_ZY_RK_INBILLDETAIL_del_dataset
(
schema: T_ZY_RK_INBILLDETAIL_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_RK_INBILLDETAIL.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataset file T_ZY_JG_PROCARTICLEDTL_del_dataset
(
schema: T_ZY_JG_PROCARTICLEDTL_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_JG_PROCARTICLEDTL.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataset file T_ZY_GH_CHANGEOWNERLIST_del_dataset
(
schema: T_ZY_GH_CHANGEOWNERLIST_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_GH_CHANGEOWNERLIST.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataset file T_ZY_GH_CHANGEOWNERDETAIL_del_dataset
(
schema: T_ZY_GH_CHANGEOWNERDETAIL_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_GH_CHANGEOWNERDETAIL.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataset file T_ZY_CK_OUTPACK_del_dataset
(
schema: T_ZY_CK_OUTPACK_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_CK_OUTPACK.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataset file T_ZY_JG_PROCMATERIALDTL_del_dataset
(
schema: T_ZY_JG_PROCMATERIALDTL_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_ZY_JG_PROCMATERIALDTL.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc select T_ZY_GH_CHANGEOWNERLIST_del_select
(
fields:
(
(fname:"DEPTID"),
(fname:"CHANGEOWNERID"),
(fname:"NEWOWNERNO"),
(fname:"NEWOWNERNAME"),
(fname:"PREOWNERNO"),
(fname:"PROOWNERNAME"),
(fname:"CHANGEDATE")
),
inputs: "T_ZY_GH_CHANGEOWNERLIST_del_dataset",
order_by:("DEPTID","CHANGEOWNERID"),
conditions:"DEPTID = '101'
AND CHANGEDATE >= TO_DATE ('2012-07-02', '%Y-%m-%d')",
cache : "true",
into: "T_ZY_GH_CHANGEOWNERLIST"
);
dataproc select T_ZY_GH_CHANGEOWNERDETAIL_del_select
(
fields:
(
(fname:"PREMANAGENUM"),
(fname:"CASE WHEN ( MANAGENUM IS NULL OR MANAGENUM = '') THEN PREMANAGENUM ELSE MANAGENUM END ",alias:"MANAGENUM",type:"string"),
(fname:"SHOPSIGNID"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREAID"),
(fname:"PRODAREANAME"),
(fname:"GRADENUM"),
(fname:"NETWEIGHT"),
(fname:"GROSSWEIGHT"),
(fname:"SHEETNUM"),
(fname:"FIRSTINDATE"),
(fname:"KINDNAME"),
(fname:"GOODSLOCID"),
(fname:"PACKNUM"),
(fname:"CHANGEOWNERNO"),
(fname:"DEPTID",alias:"DEPTID_1",type:"string"),
(fname:"GRADENUMXH"),
(fname:"SINGLESHEETNUM"),
(fname:"OWNERNAME"),
(fname:"GOODSOWNERMANAGENUM",alias:"GOODSOWNERMANAGENO",type:"string")
),
inputs: "T_ZY_GH_CHANGEOWNERDETAIL_del_dataset",
order_by:("DEPTID_1","CHANGEOWNERNO"),
cache : "true",
into: "T_ZY_GH_CHANGEOWNERDETAIL"
);
dataproc join T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_del_join
(
inputs:
(left_input:T_ZY_GH_CHANGEOWNERLIST_del_select,right_input:T_ZY_GH_CHANGEOWNERDETAIL_del_select),
join_keys:
(
("left_input.DEPTID","right_input.DEPTID_1"),
("left_input.CHANGEOWNERID","right_input.CHANGEOWNERNO")
),
into: "T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL"
);
dataproc select T_GG_SJ_GOODS_del_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"sum(GRADENUMXH)",alias:"GRADENUMXH",type:"double"),
(fname:"sum(SINGLESHEETNUM)",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_GG_SJ_GOODS_del_dataset",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE),
conditions:"GOODSSTATENO = '110'
AND R_RECORD_IS_DELETED = 0
AND DEPTID = '101'",
cache : "true",
into: "T_GG_SJ_GOODS"
);
dataproc select A_del_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"sum(GRADENUMXH)",alias:"GRADENUMXH",type:"double"),
(fname:"sum(SINGLESHEETNUM)",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_ZY_RK_INBILLDETAIL_del_dataset",
conditions:"DEPTID = '101'
AND GOODSSTATENO = '20'
AND FIRSTINDATE >= TO_DATE ('2012-07-02', '%Y-%m-%d')",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE),
into: "T_ZY_RK_INBILLDETAIL"
);
dataproc select T_ZY_RK_INBILLDETAIL_del_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"0-GRADENUMXH",alias:"GRADENUMXH",type:"double"),
(fname:"0-SINGLESHEETNUM",alias:"SINGLESHEETNUM",type:"double"),
(fname:"0-NETWEIGHT",alias:"NETWEIGHT",type:"double"),
(fname:"0-GROSSWEIGHT",alias:"GROSSWEIGHT",type:"double"),
(fname:"0-SHEETNUM",alias:"SHEETNUM",type:"double")
),
inputs: "A_del_select",
cache : "true",
into: "T_ZY_RK_INBILLDETAIL"
);
dataproc select B_del_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"0",alias:"GRADENUMXH",type:"double"),
(fname:"0",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_ZY_JG_PROCARTICLEDTL_del_dataset",
conditions:"DEPTID = '101'
AND FIRSTINDATE >= TO_DATE ('2012-07-02', '%Y-%m-%d')
AND GOODSSTATENO = '130'",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE),
into: "T_ZY_JG_PROCARTICLEDTL"
);
dataproc select T_ZY_JG_PROCARTICLEDTL_del_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"0",alias:"GRADENUMXH",type:"double"),
(fname:"0",alias:"SINGLESHEETNUM",type:"double"),
(fname:"0-NETWEIGHT",alias:"NETWEIGHT",type:"double"),
(fname:"0-GROSSWEIGHT",alias:"GROSSWEIGHT",type:"double"),
(fname:"0-SHEETNUM",alias:"SHEETNUM",type:"double")
),
inputs: "B_del_select",
cache : "true",
into: "T_ZY_JG_PROCARTICLEDTL"
);
dataproc select C_del_select
(
fields:
(
(fname:"NEWOWNERNO",alias:"OWNERID"),
(fname:"NEWOWNERNAME",alias:"OWNERNAME"),
(fname:"MANAGENUM",alias:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"0",alias:"GRADENUMXH",type:"double"),
(fname:"0",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_del_join",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE),
into: "T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL"
);
dataproc select T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_del_select
(
fields:
(
(fname:"NEWOWNERNO",alias:"OWNERID"),
(fname:"NEWOWNERNAME",alias:"OWNERNAME"),
(fname:"MANAGENUM",alias:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"0",alias:"GRADENUMXH",type:"double"),
(fname:"0",alias:"SINGLESHEETNUM",type:"double"),
(fname:"0-NETWEIGHT",alias:"NETWEIGHT",type:"double"),
(fname:"0-GROSSWEIGHT",alias:"GROSSWEIGHT",type:"double"),
(fname:"0-SHEETNUM",alias:"SHEETNUM",type:"double")
),
inputs: "C_del_select",
cache : "true",
into: "T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL"
);
dataproc select T_ZY_CK_OUTPACK_del_select
(
fields:
(
(fname:"OWNERNO"),
(fname:"OWNERNAME"),
(fname:"MANAGENUM",alias:"MANAGENO"),
(fname:"GOODSOWNERMANAGENUM",alias:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"sum(GRADENUMXH)",alias:"GRADENUMXH",type:"double"),
(fname:"sum(SINGLESHEETNUM)",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_ZY_CK_OUTPACK_del_dataset",
conditions:"DEPTID = '101'
AND OUTCUSTTIME >= TO_DATE ('2012-07-02', '%Y-%m-%d')",
group_by:(OWNERNO,OWNERNAME,MANAGENUM,GOODSOWNERMANAGENUM,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE),
cache : "true",
into: "T_ZY_CK_OUTPACK"
);
dataproc select T_GG_SJ_GOODS_del_2_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PACKNUM"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"sum(GRADENUMXH)",alias:"GRADENUMXH",type:"double"),
(fname:"sum(SINGLESHEETNUM)",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_GG_SJ_GOODS_del_dataset",
conditions:"CREATDATE >= TO_DATE ('2012-07-02', '%Y-%m-%d')",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE),
cache : "true",
into: "T_GG_SJ_GOODS"
);
dataproc select T_ZY_JG_PROCMATERIALDTL_del_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"0",alias:"GRADENUMXH",type:"double"),
(fname:"0",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_ZY_JG_PROCMATERIALDTL_del_dataset",
conditions:"vf_PROCESSID = '1'
and DEPTID = '101' ",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE),
cache : "true",
into: "T_ZY_JG_PROCMATERIALDTL"
);
dataproc select T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_del_1_select
(
fields:
(
(fname:"PREOWNERNO",alias:"OWNERID"),
(fname:"PROOWNERNAME",alias:"OWNERNAME"),
(fname:"PREMANAGENUM",alias:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"0",alias:"GRADENUMXH",type:"double"),
(fname:"0",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_del_join",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE),
cache : "true",
into: "T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL"
);
dataproc union 1_del_union
(
inputs:("T_GG_SJ_GOODS_del_select","T_ZY_RK_INBILLDETAIL_del_select","T_ZY_JG_PROCARTICLEDTL_del_select","T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_del_select","T_ZY_CK_OUTPACK_del_select","T_GG_SJ_GOODS_del_2_select","T_ZY_JG_PROCMATERIALDTL_del_select","T_ZY_GH_CHANGEOWNERLIST_T_ZY_GH_CHANGEOWNERDETAIL_del_1_select"),
into:"union_all"
);
dataproc select union_del_select
(
fields:
(
(fname:"OWNERID"),
(fname:"OWNERNAME"),
(fname:"MANAGENO"),
(fname:"GOODSOWNERMANAGENO"),
(fname:"PACKNUM"),
(fname:"GRADENUM"),
(fname:"GOODSLOCID"),
(fname:"KINDNAME"),
(fname:"SHOPSIGNNAME"),
(fname:"PRODAREANAME"),
(fname:"FIRSTINDATE"),
(fname:"sum(GRADENUMXH)",alias:"GRADENUMXH",type:"double"),
(fname:"sum(SINGLESHEETNUM)",alias:"SINGLESHEETNUM",type:"double"),
(fname:"sum(NETWEIGHT)",alias:"NETWEIGHT",type:"double"),
(fname:"sum(GROSSWEIGHT)",alias:"GROSSWEIGHT",type:"double"),
(fname:"sum(SHEETNUM)",alias:"SHEETNUM",type:"double")
),
inputs: "1_del_union",
group_by:(OWNERID,OWNERNAME,MANAGENO,GOODSOWNERMANAGENO,PACKNUM,GRADENUM,GOODSLOCID,KINDNAME,SHOPSIGNNAME,PRODAREANAME,FIRSTINDATE),
cache : "true",
into: "union_all"
);
dataproc statistics union_all_del_statistics
(
inputs:union_del_select,
table:union_all,
data_opr:delete
);
end;
start service service_2 {
"running_name" : "idx_job1",
"interval" : 0,
"volume" : 1000000000,
"threads" : 4
};
<file_sep>/complete/JINSHANG-102/job2.sql
create job 2_job(8)
begin
dataset file T_GG_SJ_GOODS_dataset
(
schema: "T_GG_SJ_GOODS_schema",
filename: "/home/natt/Data/jinshang_Data_New/T_GG_SJ_GOODS.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_GG_SJ_GOODS_syncher
(
inputs: T_GG_SJ_GOODS_dataset,
database:db,
operation:add,
table:T_GG_SJ_GOODS,
stream:true,
services :
(
(dataprocname : T_GG_SJ_GOODS_dataset,
serviceid: idx_job)
)
);
end;
run job 2_job (threads:8);
<file_sep>/processing/JINSHANG-75/init.sql
create job t_common_dept_job(t_common)
begin
dataset file t_common_dept_dataset
(
schema:t_common_dept_schema,
filename: "/home/zhaoshun/Data/jingshang_data/T_COMMON_DEPT.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc index t_common_dept_index
(
inputs: t_common_dept_dataset,
table: t_common_dept
);
dataproc doc t_common_dept_doc
(
inputs:t_common_dept_dataset,
table:t_common_dept
);
dataproc map t_common_dept_map
(
inputs:t_common_dept_dataset,
table:t_common_dept
);
end;
run job t_common_dept_job(threads:8);
create job t_gg_sj_goods_job(t_gg_sj)
begin
dataset file t_gg_sj_goods_dataset
(
schema:t_gg_sj_goods_schema,
filename:"/home/zhaoshun/Data/jingshang_data/T_GG_SJ_GOODS.txt",
serverid: 0,
splitter:(block_size: 10000000)
);
dataproc index tt_1_index
(
inputs: t_gg_sj_goods_dataset,
table: t_1
);
dataproc doc t_1_doc
(
inputs:t_gg_sj_goods_dataset,
table:t_1,
format:t_1_parser
);
end;
run job t_gg_sj_goods_job (threads:8);
<file_sep>/schema/T_SI_LOGISTICS_READY_D.sql
CREATE SCHEMA T_SI_LOGISTICS_READY_D_schema
SOURCE TYPE CSV
FIELDS(
"seg_no" type str,
"pack_num" type str,
"weight_active" type double,
"weight_theo" type double,
"length_product" type double,
"length_product_max" type double,
"width_product" type double,
"thick_product" type double,
"type_product" type str,
"n_pack" type double,
"ready_num" type str,
"order_num" type str,
"heat_num" type str,
"shopsign" type str,
"proc_mark" type str,
"time_receive" type datetime,
"aaa" type datetime,
"provider_id" type str,
"provider_name" type str
)
SCHEMA DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_SI_LOGISTICS_READY_D_parser
TYPE rcd
SCHEMA T_SI_LOGISTICS_READY_D_schema;
CREATE TABLE T_SI_LOGISTICS_READY_D using T_SI_LOGISTICS_READY_D_parser;
CREATE INDEX T_SI_LOGISTICS_READY_D_index ON TABLE T_SI_LOGISTICS_READY_D (seg_no);
<file_sep>/schema/T_ZY_RK_INBILLDETAIL.sql
create schema T_ZY_RK_INBILLDETAIL_schema
SOURCE TYPE CSV
FIELDS(
"goodsid" type str,
"deptid" type str,
"packnum" type str,
"barcode" type str,
"goodslocid" type str,
"stocktype" type str,
"ownerid" type str,
"ownername" type str,
"goodsownermanageno" type str,
"inbillid" type str,
"goodsownerpackno" type str,
"manageno" type str,
"feebegindate" type DATETIME,
"contractid" type str,
"goodsstateno" type str,
"goodsstatename" type str,
"changeownertimes" type double,
"goodstype" type str,
"goodstypename" type str,
"kindno" type str,
"kindname" type str,
"shopsignid" type str,
"shopsignname" type str,
"prodareaid" type str,
"prodareaname" type str,
"goodsbatchno" type str,
"prodstoveno" type str,
"gradenum" type str,
"gradenumthick" type double,
"gradenumwidth" type double,
"gradenumlength" type double,
"grossweight" type double,
"netweight" type double,
"poundweight" type double,
"checkweight" type double,
"sheetnum" type double,
"unit" type str,
"stockareaid" type str,
"qualitymemo" type str,
"firstinownerid" type str,
"firstindate" type DATETIME,
"outbillid" type str,
"ldglistid" type str,
"materialgoodsid" type str,
"intype" type str,
"outtype" type str,
"billstate" type double,
"outbilltime" type DATETIME,
"memo" type str,
"extsysplanobjectid" type str,
"extsyskindid" type str,
"extsysshopsignid" type str,
"extsysprodareaid" type str,
"sendflag" type double,
"r_record_create_date" type DATETIME,
"r_record_create_user" type str,
"r_record_update_date" type DATETIME,
"r_record_update_user" type str,
"r_record_is_deleted" type double,
"incusttime" type DATETIME,
"foreignlistno" type str,
"sidemark" type str,
"length_product_max" type double,
"r_record_change_flag" type double,
"sdprice" type str,
"inbillempname" type str,
"extsysgradenum" type str,
"heat_num" type str,
"errormessage" type str,
"check_type" type str,
"outdate" type DATETIME,
"yfweight" type double,
"yfsheet" type double,
"pregoodsid" type str,
"singlesheetnum" type double,
"gradenumhz" type str,
"gradenumthickhz" type double,
"singlesheet" type double,
"gradenumxh" type double,
"shipno" type str,
"shipname" type str,
"shipcd" type str,
"shiplevel" type str,
"sort_emp" type str,
"dbrkflag" type double,
"dbckflag" type double,
"dbowner" type str,
"dbgoodsid" type str,
"dispersesheet" type double,
"ply" type double,
"out_package_status" type str,
"out_package_desc" type str,
"gmpacktype" type str,
"handno" type str,
"saveno" type str,
"dangerouslevel" type str,
"acceptancecriteria" type str,
"shippingmark" type str,
"gradenumwhdw" type str,
"loclv2" type str,
"packtype" type str,
"payedflag" type str,
"collectionmanname" type str,
"loclv1" type str
)
SCHEMA DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_ZY_RK_INBILLDETAIL_parser
TYPE rcd
SCHEMA T_ZY_RK_INBILLDETAIL_schema;
CREATE TABLE T_ZY_RK_INBILLDETAIL using T_ZY_RK_INBILLDETAIL_parser;
create index T_ZY_RK_INBILLDETAIL_index on table T_ZY_RK_INBILLDETAIL(goodsid);<file_sep>/complete/JINSHANG-102/102_oracl.sql
SELECT
OWNERID,
OWNERNAME,
MANAGENO,
GOODSOWNERMANAGENO,
PACKNUM,
GRADENUM,
GOODSLOCID,
KINDNAME,
SHOPSIGNNAME,
PRODAREANAME,
FIRSTINDATE,
SUM (GRADENUMXH) GRADENUMXH,
SUM (SINGLESHEETNUM) SINGLESHEETNUM,
SUM (NETWEIGHT) NETWEIGHT,
SUM (GROSSWEIGHT) GROSSWEIGHT,
SUM (SHEETNUM) SHEETNUM
FROM
(
SELECT
OWNERID,
OWNERNAME,
MANAGENO,
GOODSOWNERMANAGENO,
PACKNUM,
GRADENUM,
GOODSLOCID,
KINDNAME,
SHOPSIGNNAME,
PRODAREANAME,
FIRSTINDATE,
SUM (GRADENUMXH) GRADENUMXH,
SUM (SINGLESHEETNUM) SINGLESHEETNUM,
SUM (NETWEIGHT) NETWEIGHT,
SUM (GROSSWEIGHT) GROSSWEIGHT,
SUM (SHEETNUM) SHEETNUM
FROM
T_GG_SJ_GOODS
WHERE
GOODSSTATENO = '110'
AND R_RECORD_IS_DELETED = 0
AND DEPTID = '101'
GROUP BY
OWNERID,
OWNERNAME,
MANAGENO,
GOODSOWNERMANAGENO,
PACKNUM,
GRADENUM,
GOODSLOCID,
KINDNAME,
SHOPSIGNNAME,
PRODAREANAME,
FIRSTINDATE
UNION ALL
SELECT
OWNERID,
OWNERNAME,
MANAGENO,
GOODSOWNERMANAGENO,
PACKNUM,
GRADENUM,
GOODSLOCID,
KINDNAME,
SHOPSIGNNAME,
PRODAREANAME,
FIRSTINDATE,
SUM (GRADENUMXH) GRADENUMXH,
SUM (SINGLESHEETNUM) SINGLESHEETNUM,
- SUM (NETWEIGHT) NETWEIGHT ,
- SUM (GROSSWEIGHT) GROSSWEIGHT ,
- SUM (SHEETNUM) SHEETNUM
FROM
T_ZY_RK_INBILLDETAIL
WHERE
DEPTID = '101'
AND GOODSSTATENO = '20'
AND FIRSTINDATE >= TO_DATE ('2011/7/2', 'YYYY-MM-DD')
GROUP BY
OWNERID,
OWNERNAME,
MANAGENO,
GOODSOWNERMANAGENO,
PACKNUM,
GRADENUM,
GOODSLOCID,
KINDNAME,
SHOPSIGNNAME,
PRODAREANAME,
FIRSTINDATE
UNION ALL
SELECT
OWNERID,
OWNERNAME,
MANAGENO,
GOODSOWNERMANAGENO,
PACKNUM,
GRADENUM,
GOODSLOCID,
KINDNAME,
SHOPSIGNNAME,
PRODAREANAME,
FIRSTINDATE,
0 GRADENUMXH,
0 SINGLESHEETNUM,
- SUM (NETWEIGHT) NETWEIGHT ,
- SUM (GROSSWEIGHT) GROSSWEIGHT ,
- SUM (SHEETNUM) SHEETNUM
FROM
T_ZY_JG_PROCARTICLEDTL
WHERE
DEPTID = '101'
AND FIRSTINDATE >= TO_DATE ('2011/7/2', 'YYYY-MM-DD')
AND GOODSSTATENO = '130'
GROUP BY
OWNERID,
OWNERNAME,
MANAGENO,
GOODSOWNERMANAGENO,
PACKNUM,
GRADENUM,
GOODSLOCID,
KINDNAME,
SHOPSIGNNAME,
PRODAREANAME,
FIRSTINDATE
UNION ALL
SELECT
NEWOWNERNO OWNERID,
NEWOWNERNAME OWNERNAME,
MANAGENUM MANAGENO,
GOODSOWNERMANAGENO,
PACKNUM,
GRADENUM,
GOODSLOCID,
KINDNAME,
SHOPSIGNNAME,
PRODAREANAME,
FIRSTINDATE,
0 GRADENUMXH,
0 SINGLESHEETNUM,
- SUM (NETWEIGHT) NETWEIGHT ,
- SUM (GROSSWEIGHT) GROSSWEIGHT ,
- SUM (SHEETNUM) SHEETNUM
FROM
(
SELECT
A .NEWOWNERNO,
A .NEWOWNERNAME,
(CASE WHEN ( MANAGENUM IS NULL OR MANAGENUM = '') THEN PREMANAGENUM ELSE MANAGENUM END ) MANAGENUM,
B.SHOPSIGNID,
B.SHOPSIGNNAME,
B.PRODAREAID,
B.PRODAREANAME,
B.GRADENUM,
B.NETWEIGHT,
B.GROSSWEIGHT,
B.SHEETNUM,
B.FIRSTINDATE,
B.KINDNAME,
B.GOODSLOCID,
B.PACKNUM,
B.GOODSOWNERMANAGENUM GOODSOWNERMANAGENO
FROM
(
SELECT
DEPTID,
CHANGEOWNERID,
NEWOWNERNO,
NEWOWNERNAME
FROM
T_ZY_GH_CHANGEOWNERLIST
WHERE
DEPTID = '101'
AND CHANGEDATE >= TO_DATE ('2012/7/2', 'YYYY-MM-DD')
) A,
T_ZY_GH_CHANGEOWNERDETAIL B
WHERE
A .CHANGEOWNERID = B.CHANGEOWNERNO
AND A .DEPTID = B.DEPTID
) TB1
GROUP BY
NEWOWNERNO,
NEWOWNERNAME,
MANAGENUM,
GOODSOWNERMANAGENO,
PACKNUM,
GRADENUM,
GOODSLOCID,
KINDNAME,
SHOPSIGNNAME,
PRODAREANAME,
FIRSTINDATE
UNION ALL
SELECT
OWNERNO,
OWNERNAME,
MANAGENUM MANAGENO,
GOODSOWNERMANAGENUM GOODSOWNERMANAGENO,
PACKNUM,
GRADENUM,
GOODSLOCID,
KINDNAME,
SHOPSIGNNAME,
PRODAREANAME,
FIRSTINDATE,
SUM (GRADENUMXH) GRADENUMXH,
SUM (SINGLESHEETNUM) SINGLESHEETNUM,
SUM (NETWEIGHT) NETWEIGHT,
SUM (GROSSWEIGHT) GROSSWEIGHT,
SUM (SHEETNUM) SHEETNUM
FROM
T_ZY_CK_OUTPACK
WHERE
DEPTID = '101'
AND OUTCUSTTIME >= TO_DATE ('2012/7/2', 'YYYY-MM-DD')
GROUP BY
OWNERNO,
OWNERNAME,
MANAGENUM,
GOODSOWNERMANAGENUM,
PACKNUM,
GRADENUM,
GOODSLOCID,
KINDNAME,
SHOPSIGNNAME,
PRODAREANAME,
FIRSTINDATE
UNION ALL
SELECT
OWNERID,
OWNERNAME,
MANAGENO,
GOODSOWNERMANAGENO,
A .PACKNUM,
GRADENUM,
GOODSLOCID,
KINDNAME,
SHOPSIGNNAME,
PRODAREANAME,
FIRSTINDATE,
SUM (GRADENUMXH) GRADENUMXH,
SUM (SINGLESHEETNUM) SINGLESHEETNUM,
SUM (NETWEIGHT) NETWEIGHT,
SUM (GROSSWEIGHT) GROSSWEIGHT,
SUM (SHEETNUM) SHEETNUM
FROM
T_GG_SJ_GOODS A,
T_ZY_CK_CLEAR B
WHERE
A .GOODSID = B.GOODSID
AND A .DEPTID = '101'
AND CREATDATE >= TO_DATE ('2012/7/2', 'YYYY-MM-DD')
GROUP BY
OWNERID,
OWNERNAME,
MANAGENO,
GOODSOWNERMANAGENO,
A .PACKNUM,
GRADENUM,
GOODSLOCID,
KINDNAME,
SHOPSIGNNAME,
PRODAREANAME,
FIRSTINDATE
UNION ALL
SELECT
OWNERID,
OWNERNAME,
MANAGENO,
GOODSOWNERMANAGENO,
PACKNUM,
GRADENUM,
GOODSLOCID,
KINDNAME,
SHOPSIGNNAME,
PRODAREANAME,
FIRSTINDATE,
0 GRADENUMXH,
0 SINGLESHEETNUM,
SUM (NETWEIGHT) NETWEIGHT,
SUM (GROSSWEIGHT) GROSSWEIGHT,
SUM (SHEETNUM) SHEETNUM
FROM
T_ZY_JG_PROCMATERIALDTL
WHERE
DEPTID = '101'
AND EXISTS (
SELECT
1
FROM
T_ZY_JG_PROCESS
WHERE
PROCESSID = T_ZY_JG_PROCMATERIALDTL.PROCESSID
AND DEPTID = T_ZY_JG_PROCMATERIALDTL.DEPTID
AND OUTCARBILLTIME >= TO_DATE ('2012/7/2', 'YYYY-MM-DD')
)
GROUP BY
OWNERID,
OWNERNAME,
MANAGENO,
GOODSOWNERMANAGENO,
PACKNUM,
GRADENUM,
GOODSLOCID,
KINDNAME,
SHOPSIGNNAME,
PRODAREANAME,
FIRSTINDATE
UNION ALL
SELECT
PREOWNERNO OWNERID,
PROOWNERNAME OWNERNAME,
PREMANAGENUM MANAGENO,
GOODSOWNERMANAGENO,
PACKNUM,
GRADENUM,
GOODSLOCID,
KINDNAME,
SHOPSIGNNAME,
PRODAREANAME,
FIRSTINDATE,
0 GRADENUMXH,
0 SINGLESHEETNUM,
SUM (NETWEIGHT) NETWEIGHT,
SUM (GROSSWEIGHT) GROSSWEIGHT,
SUM (SHEETNUM) SHEETNUM
FROM
(
SELECT
A .PREOWNERNO,
A .PROOWNERNAME,
B.PREMANAGENUM,
B.NETWEIGHT,
B.GROSSWEIGHT,
B.SHEETNUM,
B.SHOPSIGNID,
B.SHOPSIGNNAME,
B.PRODAREAID,
B.PRODAREANAME,
B.GRADENUM,
B.FIRSTINDATE,
B.KINDNAME,
B.GOODSLOCID,
B.PACKNUM,
B.PREGOODSOWNERMANAGENUM GOODSOWNERMANAGENO
FROM
(
SELECT
DEPTID,
CHANGEOWNERID,
PREOWNERNO,
PROOWNERNAME
FROM
T_ZY_GH_CHANGEOWNERLIST
WHERE
DEPTID = '101'
AND CHANGEDATE >= TO_DATE ('2012/7/2', 'YYYY-MM-DD')
) A,
T_ZY_GH_CHANGEOWNERDETAIL B
WHERE
A .CHANGEOWNERID = B.CHANGEOWNERNO
AND A .DEPTID = B.DEPTID
) TB1
GROUP BY
PREOWNERNO,
PROOWNERNAME,
PREMANAGENUM,
GOODSOWNERMANAGENO,
PACKNUM,
GRADENUM,
GOODSLOCID,
KINDNAME,
SHOPSIGNNAME,
PRODAREANAME,
FIRSTINDATE
) TBX
WHERE
OWNERID = 'KH05821'
AND NETWEIGHT <> 0
GROUP BY
OWNERID,
OWNERNAME,
MANAGENO,
GOODSOWNERMANAGENO,
PACKNUM,
GRADENUM,
GOODSLOCID,
KINDNAME,
SHOPSIGNNAME,
PRODAREANAME,
FIRSTINDATE
<file_sep>/uncomplete/JINSHANG-97/schema/T_ZY_GH_CHANGEOWNERLIST.sql
create database db;
use db;
create schema t_zy_gh_changeownerlist_schema
source type CSV
fields(
"changeownerid" type str,
"deptid" type str,
"proownername" type str,
"preownerno" type str,
"contractno" type str,
"premanagenum" type str,
"newownername" type str,
"newownerno" type str,
"newmanagenum" type str,
"changedate" type datetime format '%Y/%m/%d %H:%M:%S',
"getbiilldate" type datetime format '%Y/%m/%d %H:%M:%S',
"foreignlistno" type str,
"orderlistno" type str,
"outcarbilltime" type datetime format '%Y/%m/%d %H:%M:%S',
"outcarbillempno" type str,
"outcarbillempname" type str,
"outcarbillmemo" type str,
"r_record_create_date" type datetime format '%Y/%m/%d %H:%M:%S',
"r_record_create_user" type str,
"r_record_update_date" type datetime format '%Y/%m/%d %H:%M:%S',
"r_record_update_user" type str,
"r_record_is_deleted" type double,
"netweightsum" type double,
"grossweightsum" type double,
"sheetnumsum" type double,
"originsettle" type double,
"r_record_change_flag" type double,
"issplit" type double,
"outpassword" type str,
"invoicekindid" type str,
"invoicekindname" type str,
"sortemp" type str,
"sortemptele" type str,
"settlestatus" type str,
"confirm_date" type datetime format '%Y/%m/%d %H:%M:%S',
"confirm_emp" type str,
"confirm_flag" type str default value '0',
"dtlcount" type double,
"issettled" type double,
"jfsendflag" type double,
"jyzxflag" type double,
"sendmessage" type str,
"jyzxcheckdate" type datetime format '%Y/%m/%d %H:%M:%S',
"jyzxcheckflag" type double,
"idcode" type str,
"username" type str
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER t_zy_gh_changeownerlist_parser
TYPE rcd
SCHEMA t_zy_gh_changeownerlist_schema;
<file_sep>/complete/JINSHANG-110/jinshang-110.sql
FOR dtl in
(SELECT sum(STORE_AMOUNT) STORE_AMOUNT,sum(STORE_IN_AMOUNT) STORE_IN_AMOUNT, sum(STORE_OUT_AMOUNT) STORE_OUT_AMOUNT,sum(STORE_PROCESS_AMOUNT) STORE_PROCESS_AMOUNT
FROM
( SELECT /+index(t T_GG_SJ_GOODS_INDX05)/sum(t.netweight) STORE_AMOUNT,0 STORE_IN_AMOUNT,0 STORE_OUT_AMOUNT,0 STORE_PROCESS_AMOUNT
FROM wmsdba.t_gg_sj_goods@wms t
WHERE goodsstateno = '20'
AND (deptid NOT LIKE '03%'
AND deptid NOT LIKE 'ZZ%'
AND deptid NOT LIKE 'MG%')
UNION ALL --RK
SELECT 0 STORE_AMOUNT,sum(t.netweightsum) STORE_IN_AMOUNT,0 STORE_OUT_AMOUNT,0 STORE_PROCESS_AMOUNT
FROM wmsdba.t_zy_rk_inbill@wms t
WHERE r_record_create_date >= trunc(sysdate - 1) + 14 / 24
AND r_record_create_date < trunc(sysdate) + 14 / 24
AND (deptid NOT LIKE '03%'
AND deptid NOT LIKE 'ZZ%'
AND deptid NOT LIKE 'MG%')
UNION ALL --CK
SELECT 0 STORE_AMOUNT,0 STORE_IN_AMOUNT,sum(t.netweightsum) STORE_OUT_AMOUNT,0 STORE_PROCESS_AMOUNT
FROM wmsdba.t_zy_ck_outbill@wms t
WHERE r_record_create_date >= trunc(sysdate - 1) + 14 / 24
AND r_record_create_date < trunc(sysdate) + 14 / 24
AND (deptid NOT LIKE '03%'
AND deptid NOT LIKE 'ZZ%'
AND deptid NOT LIKE 'MG%')
UNION ALL --JG
SELECT 0 STORE_AMOUNT,0 STORE_IN_AMOUNT,0 STORE_OUT_AMOUNT,sum(t.preweightsum) STORE_PROCESS_AMOUNT
FROM wmsdba.t_zy_jg_process@wms t
WHERE r_record_create_date >= trunc(sysdate - 1) + 14 / 24
AND r_record_create_date < trunc(sysdate) + 14 / 24
AND (deptid NOT LIKE '03%'
AND deptid NOT LIKE 'ZZ%'
AND deptid NOT LIKE 'MG%'))) LOOP
INSERT INTO t_statistics_warehouse(STORE_AMOUNT,STORE_IN_AMOUNT,STORE_OUT_AMOUNT,STORE_PROCESS_AMOUNT)
VALUES (dtl.STORE_AMOUNT,
dtl.STORE_IN_AMOUNT,
dtl.STORE_OUT_AMOUNT,
dtl.STORE_PROCESS_AMOUNT) ;
COMMIT;
END LOOP;
<file_sep>/schema/T_BM_UI_CONFIGURE.sql
create schema T_BM_UI_CONFIGURE_schema
source type CSV
fields(
"manage_no" type str,
"deptid" type str,
"configure_no" type str,
"configure_desc" type str,
"configure_grade" type str,
"configure_node_no" type str,
"cf_value1" type str,
"cf_value2" type str,
"cf_value3" type double,
"cf_value4" type double,
"configure_remark" type str,
"r_record_create_date" type DATETIME,
"r_record_create_user" type str,
"r_record_update_date" type DATETIME,
"r_record_update_user" type str,
"r_record_is_deleted" type double,
"r_record_change_flag" type double,
"cf_value5" type DATETIME
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "NULL";
CREATE PARSER T_BM_UI_CONFIGURE_parser
TYPE rcd
SCHEMA T_BM_UI_CONFIGURE_schema;
CREATE TABLE T_BM_UI_CONFIGURE using T_BM_UI_CONFIGURE_parser;
CREATE INDEX T_BM_UI_CONFIGURE_index ON TABLE T_BM_UI_CONFIGURE (manage_no);<file_sep>/uncomplete/JINSHANG-99/jinshang-99.sql
SELECT pp.STOCKFLAG,
INWeight,
OUTWeight,
ChangeOwnerWeight,
KCWeight,
KCSheetnum,
js
FROM
(SELECT STOCKFLAG,
SUM(INWeight) INWeight,
SUM(OUTWeight) OUTWeight,
SUM(KCWeight) KCWeight,
SUM(KCSheetnum) KCSheetnum,
SUM(js) js
FROM
(SELECT tb1.*,
tb2.netweight INWeight,
tb3.netweight OUTWeight,
tb5.netweight KCWeight,
tb5.sheetnum KCSheetnum,
tb5.js js
FROM
(SELECT goodsid,
substr(goodslocid,1,1) stockflag
FROM t_gg_sj_goods
WHERE deptid='0301') tb1
LEFT JOIN
(SELECT goodsid,
netweight
FROM t_zy_rk_inbilldetail
WHERE deptid='0301'
AND goodsstateno='20'
AND firstindate<TO_DATE('2015/8/4','yyyy-mm-dd')
AND firstindate>=to_date('2015/7/3','yyyy-mm-dd') )tb2 ON tb1.goodsid=tb2.goodsid
LEFT JOIN
(SELECT goodsid,
netweight
FROM t_zy_ck_outpack
WHERE deptid='0301'
AND OutCustTime<to_date('2015/8/4','yyyy-mm-dd')
AND OutCustTime>=to_date('2015/7/3','yyyy-mm-dd') )tb3 ON tb1.goodsid=tb3.goodsid
LEFT JOIN
( SELECT goodsid,
sum(netweight) AS netweight,
sum(js) js,
sum(sheetnum) sheetnum
FROM
(SELECT goodsid,
netweight,
sheetnum,
1 js
FROM t_gg_sj_goods
WHERE deptid='0301'
AND goodsstateno='20'
AND R_RECORD_IS_DELETED=0
UNION ALL SELECT goodsid,-netweight,-sheetnum,
-1 js
FROM t_zy_rk_inbilldetail
WHERE deptid='0301'
AND (intype='20'
OR intype IS NULL)
AND firstindate>=to_date('2015/8/4','yyyy-mm-dd')
AND goodsstateno='20'
UNION ALL SELECT goodsid,-netweight,-sheetnum,
-1 js
FROM T_GG_SJ_Goods
WHERE deptid='0301'
AND intype='60'
AND firstindate>=to_date('2015/8/4','yyyy-mm-dd')
UNION ALL SELECT goodsid,
netweight,
sheetnum,
1 js
FROM t_zy_ck_outpack
WHERE deptid='0301'
AND goodsstateno IN ('40',
'30')
AND OutCustTime>=to_date('2015/8/4','yyyy-mm-dd')
UNION ALL SELECT PROCMATERIALDTLID,
netweight,
sheetnum,
1 js
FROM T_ZY_JG_ProcMaterialDtl
WHERE deptid='0301'
AND r_record_create_date>=to_date('2015/8/4','yyyy-mm-dd')) tbx
GROUP BY goodsid) tb5 ON tb1.goodsid=tb5.goodsid ) ttt
WHERE NOT (INWeight IS NULL
AND OUTWeight IS NULL
AND KCWeight = 0
AND KCSheetnum = 0
AND js = 0)
GROUP BY STOCKFLAG) pp
LEFT JOIN
( SELECT substr(goodslocid,1,1)STOCKFLAG ,
sum(netweight) ChangeOwnerWeight
FROM t_zy_gh_changeownerdetail
WHERE deptid='0301'
AND r_record_create_date<TO_DATE('2015/8/4','yyyy-mm-dd')
AND r_record_create_date>=to_date('2015/7/3','yyyy-mm-dd')
GROUP BY substr(goodslocid,1,1)) GH ON pp.STOCKFLAG=GH.STOCKFLAG;
<file_sep>/schema/T_GU_GUB.sql
create schema T_GU_GUB_schema
source type CSV
fields(
"gubillid" type str,
"deptid" type str,
"ownerid" type str,
"lenderid" type str,
"gutype" type str,
"sysbillid" type str,
"extbillid" type str,
"preenddate" type DATETIME,
"gubillstate" type str,
"weightsum" type double,
"sheetnumsum" type double,
"gubillmemo" type str,
"r_record_is_deleted" type double,
"r_record_create_user" type str,
"r_record_create_date" type DATETIME,
"r_record_update_user" type str,
"r_record_update_date" type DATETIME,
"r_record_change_flag" type double,
"createdman" type str,
"ownername" type str,
"importfrom" type str,
"lendername" type str,
"releaseweight" type double,
"releasesheetnum" type double
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "NULL";
CREATE PARSER T_GU_GUB_parser
TYPE rcd
SCHEMA T_GU_GUB_schema;
CREATE TABLE T_GU_GUB using T_GU_GUB_parser;
CREATE INDEX T_GU_GUB_index ON TABLE T_GU_GUB (gubillid);<file_sep>/complete/JINSHANG-75/jinshang-75.sql
SELECT
NVL (GOODSID, 'null') AS GOODSID,
NVL (DEPTID, 'null') AS DEPTID,
(
SELECT
deptname
FROM
t_common_dept D
WHERE
D .deptid = G .deptid
) AS DEPTNAME,
NVL (BARCODE, 'null') AS BARCODE,
NVL (GOODSLOCID, 'null') AS GOODSLOCID,
NVL (
DECODE (
STOCKTYPE,
'004001',
'室内库',
'004002',
'室外库',
NULL,
'室内库'
),
'null'
) AS STOCKTYPE,
NVL (OWNERID, 'null') AS OWNERID,
NVL (OWNERNAME, 'null') AS OWNERNAME,
NVL (GOODSOWNERMANAGENO, 'null') AS GOODSOWNERMANAGENO,
NVL (INBILLID, 'null') AS INBILLID,
NVL (PACKNUM, 'null') AS PACKNUM,
NVL (GOODSOWNERPACKNO, 'null') AS GOODSOWNERPACKNO,
NVL (MANAGENO, 'null') AS MANAGENO,
NVL (
TO_CHAR (
FEEBEGINDATE,
'YYYY-MM-DD hh24:mi:ss'
),
'null'
) AS FEEBEGINDATE,
NVL (CONTRACTID, 'null') AS CONTRACTID,
NVL (GOODSSTATENO, 'null') AS GOODSSTATENO,
NVL (GOODSSTATENAME, 'null') AS GOODSSTATENAME,
NVL (
TO_CHAR (CHANGEOWNERTIMES),
'null'
) AS CHANGEOWNERTIMES,
NVL (GOODSTYPE, 'null') AS GOODSTYPE,
NVL (GOODSTYPENAME, 'null') AS GOODSTYPENAME,
NVL (KINDNO, 'null') AS KINDNO,
NVL (KINDNAME, 'null') AS KINDNAME,
NVL (SHOPSIGNID, 'null') AS SHOPSIGNID,
NVL (SHOPSIGNNAME, 'null') AS SHOPSIGNNAME,
NVL (PRODAREAID, 'null') AS PRODAREAID,
NVL (PRODAREANAME, 'null') AS PRODAREANAME,
NVL (GOODSBATCHNO, 'null') AS GOODSBATCHNO,
NVL (PRODSTOVENO, 'null') AS PRODSTOVENO,
NVL (GRADENUM, 'null') AS GRADENUM,
NVL (GRADENUMTHICK, 0) AS GRADENUMTHICK,
NVL (
TO_CHAR (GRADENUMWIDTH),
'null'
) AS GRADENUMWIDTH,
NVL (
TO_CHAR (GRADENUMLENGTH),
'null'
) AS GRADENUMLENGTH,
NVL (GROSSWEIGHT, 0) AS GROSSWEIGHT,
NVL (NETWEIGHT, 0) AS NETWEIGHT,
NVL (POUNDWEIGHT, 0) AS POUNDWEIGHT,
NVL (CHECKWEIGHT, 0) AS CHECKWEIGHT,
NVL (SHEETNUM, 0) AS SHEETNUM,
NVL (UNIT, 'null') AS UNIT,
NVL (PACKTYPE, 'null') AS PACKTYPE,
NVL (STOCKAREAID, 'null') AS STOCKAREAID,
NVL (QUALITYMEMO, 'null') AS QUALITYMEMO,
NVL (FIRSTINOWNERID, 'null') AS FIRSTINOWNERID,
NVL (
TO_CHAR (
FIRSTINDATE,
'YYYY-MM-DD hh24:mi:ss'
),
'null'
) AS FIRSTINDATE,
NVL (OUTBILLID, 'null') AS OUTBILLID,
NVL (LDGLISTID, 'null') AS LDGLISTID,
NVL (MATERIALGOODSID, 'null') AS MATERIALGOODSID,
NVL (
DECODE (
INTYPE,
'20',
'正常入库',
'50',
'退货入库',
'99',
'其它'
),
'null'
) AS INTYPE,
NVL (OUTTYPE, 'null') AS OUTTYPE,
NVL (TO_CHAR(BILLSTATE), 'null') AS BILLSTATE,
NVL (
TO_CHAR (
OUTBILLTIME,
'YYYY-MM-DD hh24:mi:ss'
),
'null'
) AS OUTBILLTIME,
NVL (MEMO, 'null') AS MEMO,
NVL (SDPRICE, 'null') AS SDPRICE,
NVL (SIDEMARK, 'null') AS TRUCKNO,
NVL (
TO_CHAR (
OUTBILLTIME,
'YYYY-MM-DD hh24:mi:ss'
),
'null'
) AS OUTCUSTTIME,
NVL (
TO_CHAR (ROUND(SYSDATE - FIRSTINDATE)),
'null'
) AS STORAGEDAY,
NVL (
TO_CHAR (LENGTH_PRODUCT_MAX),
'null'
) AS LENGTHMAX
FROM
t_gg_sj_goods G
WHERE
1 = 1
AND GOODSID = 'ww'
AND PACKNUM = 'ww'
AND deptId = 'ww'
<file_sep>/complete/JINSHANG-89&90/jinshang-90.sql
SELECT prodareaname,
sum(netweightsum)
FROM t_gg_sj_goods
WHERE goodsstateno=20
AND (deptid NOT LIKE '03%'
AND deptid NOT LIKE 'ZZ%'
AND deptid NOT LIKE 'MG%')
GROUP BY prodareaname;
<file_sep>/uncomplete/JINSHANG-104&106/jinshang-104.sql
SELECT kindname,
sum(netweight) netweight,
round(avg(sysdate-firstindate),0) avgday
FROM t_gg_sj_goods t
WHERE deptid='0106'
AND goodsstateno=20
AND EXISTS
(SELECT 1
FROM WL.T_OWNER W
WHERE SEG_NO='00103'
AND T.DEPTID=W.DEPTID
AND t.ownerid=w.owner_id)
GROUP BY kindname;
<file_sep>/uncomplete/JINSHANG-79/jinshang-79.sql
SELECT to_char(sysdate,'yyyy-mm-dd hh24')||':00',
sum(a),
sum(b),
sum(c)
FROM
( SELECT sum(netweightsum) a,
0 b,
0 c
FROM t_zy_rk_inbill
WHERE r_record_create_date>sysdate-1/24
UNION ALL SELECT 0 a,
sum(netweightsum) b,
0 c
FROM t_zy_ck_outbill
WHERE r_record_create_date>sysdate-1/24
UNION ALL SELECT 0 a,
0 b,
sum(netweightsum) c
FROM t_zy_gh_changeownerlist
WHERE r_record_create_date>sysdate-1/24);
<file_sep>/complete/JINSHANG-75/jql查询语句.sql
SELECT Nvl(GOODSID, 'null') AS GOODSID,
Nvl(DEPTID, 'null') AS DEPTID,
VF_DEPTNAME AS DEPTNAME,
Nvl(BARCODE, 'null') AS BARCODE,
Nvl(GOODSLOCID, 'null') AS GOODSLOCID,
Nvl(case INTYPE when '20' then '正常入库' when '50' then '退货入库' when '99' then '其它' end,'null') AS STOCKTYPE,
Nvl(OWNERID, 'null') AS OWNERID,
Nvl(OWNERNAME, 'null') AS OWNERNAME,
Nvl(GOODSOWNERMANAGENO, 'null') AS GOODSOWNERMANAGENO,
Nvl(INBILLID, 'null') AS INBILLID,
Nvl(PACKNUM, 'null') AS PACKNUM,
Nvl(GOODSOWNERPACKNO, 'null') AS GOODSOWNERPACKNO,
Nvl(MANAGENO, 'null') AS MANAGENO,
Nvl(CONTRACTID, 'null') AS CONTRACTID,
Nvl(GOODSSTATENO, 'null') AS GOODSSTATENO,
Nvl(GOODSSTATENAME, 'null') AS GOODSSTATENAME,
CHANGEOWNERTIMES AS CHANGEOWNERTIMES,
Nvl(GOODSTYPE, 'null') AS GOODSTYPE,
Nvl(GOODSTYPENAME, 'null') AS GOODSTYPENAME,
Nvl(KINDNO, 'null') AS KINDNO,
Nvl(KINDNAME, 'null') AS KINDNAME,
Nvl(SHOPSIGNID, 'null') AS SHOPSIGNID,
Nvl(SHOPSIGNNAME, 'null') AS SHOPSIGNNAME,
Nvl(PRODAREAID, 'null') AS PRODAREAID,
Nvl(PRODAREANAME, 'null') AS PRODAREANAME,
Nvl(GOODSBATCHNO, 'null') AS GOODSBATCHNO,
Nvl(PRODSTOVENO, 'null') AS PRODSTOVENO,
Nvl(GRADENUM, 'null') AS GRADENUM,
Nvl(GRADENUMTHICK, 0) AS GRADENUMTHICK,
Nvl(GROSSWEIGHT, 0) AS GROSSWEIGHT,
Nvl(NETWEIGHT, 0) AS NETWEIGHT,
Nvl(POUNDWEIGHT, 0) AS POUNDWEIGHT,
Nvl(CHECKWEIGHT, 0) AS CHECKWEIGHT,
Nvl(SHEETNUM, 0) AS SHEETNUM,
Nvl(UNIT, 'null') AS UNIT,
Nvl(PACKTYPE, 'null') AS PACKTYPE,
Nvl(STOCKAREAID, 'null') AS STOCKAREAID,
Nvl(QUALITYMEMO, 'null') AS QUALITYMEMO,
Nvl(FIRSTINOWNERID, 'null') AS FIRSTINOWNERID,
Nvl(OUTBILLID, 'null') AS OUTBILLID,
Nvl(LDGLISTID, 'null') AS LDGLISTID,
Nvl(MATERIALGOODSID, 'null') AS MATERIALGOODSID,
Nvl(case STOCKTYPE when '004001' then '室内库' when '004002' then '室外库' when 'NULL' then '室内库' end, 'null') AS INTYPE,
Nvl(OUTTYPE, 'null') AS OUTTYPE,
Nvl(BILLSTATE, 'null') AS BILLSTATE,
Nvl(MEMO, 'null') AS MEMO,
Nvl(SDPRICE, 'null') AS SDPRICE,
Nvl(SIDEMARK, 'null') AS TRUCKNO
FROM t_gg_sj_goods;
<file_sep>/schema/T_ZY_JS_SETTLEMENTDETAIL.sql
create schema T_ZY_JS_SETTLEMENTDETAIL_schema
SOURCE TYPE CSV
FIELDS(
"manageno" type str,
"deptid" type str,
"settleno" type str,
"settlecd" type str,
"goodsid" type str,
"packnum" type str,
"node" type str,
"nodename" type str,
"businesstype" type str,
"businesstypename" type str,
"businessno" type str,
"feetype" type str,
"feetypename" type str,
"settleformula" type str,
"countunit" type double,
"settlenum" type double,
"settlesum" type double,
"unit" type str,
"weight" type double,
"grossweight" type double,
"generatedate" type DATETIME,
"priceno" type str,
"state" type double,
"deptno" type str,
"deptname" type str,
"ownerno" type str,
"ownername" type str,
"resourceno" type str,
"pregoodsid" type str,
"memo" type str,
"sendflag" type double,
"r_record_create_date" type DATETIME,
"r_record_create_user" type str,
"r_record_update_date" type DATETIME,
"r_record_update_user" type str,
"r_record_is_deleted" type double,
"invoicekindid" type str,
"invoicekindname" type str,
"r_record_change_flag" type double,
"settle_contract_id" type str,
"settle_contract_no" type str,
"sub_biz_type_id" type str,
"sub_biz_type_name" type str,
"first_in_date" type DATETIME,
"out_store_date" type DATETIME,
"free_days" type double,
"receive_fee" type double,
"jgbusinessno" type str,
"paytype" type str,
"billempname" type str,
"firstcountunit" type double,
"addfeelink" type str,
"settlelock" type str,
"jyzxflag" type double,
"foreignlistno" type str,
"yts" type double,
"mts" type double,
"direction" type str,
"settlesumused" type double,
"settledeptname" type str,
"kpamount" type double,
"username" type str,
"settlesumtax" type double,
"settlesumnotax" type double,
"cbcountunit" type double,
"cbsettlesum" type double,
"dtlinvoiceno" type str,
"invoicenodate" type str,
"dtlinvoicetypename" type str
)
SCHEMA DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_ZY_JS_SETTLEMENTDETAIL_parser
TYPE rcd
SCHEMA T_ZY_JS_SETTLEMENTDETAIL_schema;
CREATE TABLE T_ZY_JS_SETTLEMENTDETAIL using T_ZY_JS_SETTLEMENTDETAIL_parser;
create index T_ZY_JS_SETTLEMENTDETAIL_index on table T_ZY_JS_SETTLEMENTDETAIL(manageno);<file_sep>/complete/JINSHANG-84/jinshang-84.sql
SELECT cl.lock_flag,sum(net_weight),COUNT *
FROM wmsdba.t_tm_check_goods_log cl
WHERE sys_id = 'STM06'
AND CREATE_DATE>to_date(to_char(sysdate,'yyyy-mm-dd'),'yyyy-mm-dd')
GROUP BY cl.lock_flag;
<file_sep>/schema/T_ZY_JG_PROCMATERIALDTL.sql
create schema T_ZY_JG_PROCMATERIALDTL_schema
SOURCE TYPE CSV
FIELDS(
"procmaterialdtlid" type str,
"deptid" type str,
"processid" type str,
"packnum" type str,
"goodsid" type str,
"barcode" type str,
"goodslocid" type str,
"ownerid" type str,
"ownername" type str,
"goodsownermanageno" type str,
"goodsownerpackno" type str,
"manageno" type str,
"feebegindate" type datetime,
"contractid" type str,
"goodsstateno" type str,
"goodsstatename" type str,
"changeownertimes" type double,
"goodstype" type str,
"goodstypename" type str,
"kindno" type str,
"kindname" type str,
"shopsignid" type str,
"shopsignname" type str,
"prodareaid" type str,
"prodareaname" type str,
"goodsbatchno" type str,
"prodstoveno" type str,
"gradenum" type str,
"gradenumthick" type double,
"gradenumwidth" type double,
"gradenumlength" type double,
"grossweight" type double,
"netweight" type double,
"poundweight" type double,
"checkweight" type double,
"sheetnum" type double,
"packtype" type str,
"packtypename" type str,
"unit" type str,
"qualitymemo" type str,
"firstinownerid" type str,
"firstindate" type datetime,
"inbillid" type str,
"intype" type str,
"r_record_create_date" type datetime,
"r_record_create_user" type str,
"r_record_update_date" type datetime,
"r_record_update_user" type str,
"r_record_is_deleted" type double,
"orderby" type double,
"r_record_change_flag" type double,
"shopsigncd" type str,
"customerid" type str,
"chanxian" type str,
"ply" type double,
"memo" type str,
"jyzxflag" type double,
"loclv1" type str,
"loclv2" type str,
"specialmemo" type str
)
SCHEMA DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_ZY_JG_PROCMATERIALDTL_parser
TYPE rcd
SCHEMA T_ZY_JG_PROCMATERIALDTL_schema;
CREATE TABLE T_ZY_JG_PROCMATERIALDTL using T_ZY_JG_PROCMATERIALDTL_parser;
create index T_ZY_JG_PROCMATERIALDTL_index on table T_ZY_JG_PROCMATERIALDTL(procmaterialdtlid);
<file_sep>/schema/T_ZY_RK_INBILL.sql
create schema T_ZY_RK_INBILL_schema
SOURCE TYPE CSV
FIELDS(
"inbillid" type str,
"deptid" type str,
"foreignlistno" type str,
"goodsbatchno" type str,
"intype" type str,
"feebegindate" type DATETIME,
"incusttime" type DATETIME,
"inbillownername" type str,
"inbillownerno" type str,
"inbillempno" type str,
"inbillempname" type str,
"inbilltime" type DATETIME,
"inbillmemo" type str,
"kindno" type str,
"kindname" type str,
"shopsignname" type str,
"prodareaname" type str,
"prodareaid" type str,
"remanageno" type str,
"shopsignid" type str,
"truckgroupname" type str,
"truckgroupno" type str,
"planno" type str,
"manageno" type str,
"sheetnumsum" type double,
"grossweightsum" type double,
"netweightsum" type double,
"goodsownermanageno" type str,
"firstindate" type DATETIME,
"gradenum" type str,
"r_record_create_date" type DATETIME,
"r_record_create_user" type str,
"r_record_update_date" type DATETIME,
"r_record_update_user" type str,
"r_record_is_deleted" type double,
"stocktype" type str,
"r_record_change_flag" type double,
"rktype" type str,
"trans_type" type str,
"finish_date" type DATETIME,
"check_date" type DATETIME,
"check_type" type str,
"sort_emp" type str,
"check_emp" type str,
"loclv1" type str,
"loclv2" type str,
"dcemp" type str,
"qzemp" type str,
"dcemp2" type str,
"sendmessage" type str,
"dtlcount" type double,
"sidemark" type str,
"ischecked" type double,
"sheetsumsum" type double,
"issettled" type double,
"handno" type str,
"saveno" type str,
"deptfrom" type str,
"jfsendflag" type double,
"checkdtlcount" type double
)
SCHEMA DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_ZY_RK_INBILL_parser
TYPE rcd
SCHEMA T_ZY_RK_INBILL_schema;
CREATE TABLE T_ZY_RK_INBILL using T_ZY_RK_INBILL_parser;
create index T_ZY_RK_INBILL_index on table T_ZY_RK_INBILL(inbillid);<file_sep>/schema/T_TM_CHECK_GOODS_LOG.sql
CREATE SCHEMA T_TM_CHECK_GOODS_LOG_schema
SOURCE TYPE CSV
FIELDS(
"request_sn" type str,
"sys_id" type str,
"dept_id" type str,
"owner_id" type str,
"resource_num" type str,
"pack_num" type str,
"goods_id" type str,
"act_type" type str,
"net_weight" type double,
"gross_weight" type double,
"create_date" type datetime,
"business_type" type str,
"stm_good_id" type str,
"spec" type str,
"wprovider_id" type str,
"wprovider_name" type str,
"owner_name" type str,
"provider_id" type str,
"provider_name" type str,
"kindname" type str,
"shopsignname" type str,
"prodareaname" type str,
"gradenumthick" type double,
"gradenumwidth" type double,
"gradenumlength" type double,
"lock_flag" type str,
"result_message" type str,
"matching_rules" type str,
"used_time" type double,
"wms_resource_num" type str,
"wms_pack_num" type str,
"goodslocid" type str,
"barcode" type str,
"wms_goods_id" type str,
"modi_date" type datetime,
"remark" type str,
"access_plat" type str,
"goods_owner_id" type str,
"vas_member_id" type str,
"kind_no" type str,
"plan_qty" type double,
"rule_type" type str,
"check_rules" type str,
"wms_net_weight" type double,
"wms_qty" type double,
"pack_flag" type str
)
SCHEMA DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_TM_CHECK_GOODS_LOG_parser
TYPE rcd
SCHEMA T_TM_CHECK_GOODS_LOG_schema;
CREATE TABLE T_TM_CHECK_GOODS_LOG using T_TM_CHECK_GOODS_LOG_parser;
CREATE INDEX T_TM_CHECK_GOODS_LOG_index ON TABLE T_TM_CHECK_GOODS_LOG (request_sn);
<file_sep>/processing/JINSHANG-76/jinshang-76.sql
SELECT *
FROM
( SELECT rownum AS rownum_,
aa.*
FROM
( SELECT /*+ index(g,T_GG_SJ_GOODS_INDX02) */ nvl (DEPTID, 'null') AS DEPTID,
( SELECT deptname
FROM wmsdba.t_common_dept d
WHERE d.deptid = g.deptid ) AS DEPTNAME,
nvl (GRADENUM, 'null') AS GRADENUM,
nvl (SHOPSIGNID, 'null') AS SHOPSIGNID,
nvl (SHOPSIGNNAME, 'null') AS SHOPSIGNNAME,
nvl (PRODAREAID, 'null') AS PRODAREAID,
nvl (PRODAREANAME, 'null') AS PRODAREANAME,
nvl (KINDNO, 'null') AS KINDNO,
nvl (KINDNAME, 'null') AS KINDNAME,
nvl (ownername, 'null') AS OWNERSHORT,
nvl (GOODSOWNERPACKNO, 'null') AS GOODSOWNERPACKNO,
nvl (SUM(NETWEIGHT), 0) AS NETWEIGHT,
nvl (SUM(GROSSWEIGHT), 0) AS GROSSWEIGHT,
nvl (SUM(SHEETNUM), 0) AS SHEETNUM
FROM wmsdba.t_gg_sj_goods g
WHERE 1 = 1
AND ( DEPTID LIKE DECODE (
( SELECT COUNT(1)
FROM wmsdba.t_bm_ui_configure c
WHERE c.configure_no = 'COMM'
AND DEPTID = '0301' ), 0, substr('0301', 0, 2) || '%','0301' ) )
AND goodsstateno = '20'
AND KINDNAME LIKE '%' || '²»Ðâ¸ÖÏß²Ä' || '%'
AND SHOPSIGNNAME LIKE '%' || 'MR T-2.5BA' || '%'
AND GRADENUM LIKE '%' || '11' || '%'
AND PRODAREANAME LIKE '%' || 'ºª¸Ö' || '%'
GROUP BY g.deptid,
GRADENUM,
SHOPSIGNID,
SHOPSIGNNAME,
PRODAREAID,
PRODAREANAME,
KINDNO,
KINDNAME,
ownername,
GOODSOWNERPACKNO ) aa )
WHERE rownum_ > 0
AND rownum_ <= 10<file_sep>/complete/JINSHANG-102/zidonghua.sql
t_zy_ck_clear_insert_q1=begin
dataset file T_ZY_CK_CLEAR1_1_dataset
(
schema: "T_ZY_CK_CLEAR_schema",
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_ck_clear/insert/insert.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_CK_CLEAR_syncher
(
inputs: T_ZY_CK_CLEAR1_1_dataset,
database:db,
operator:add,
table:T_ZY_CK_CLEAR
);
end
t_zy_jg_process_insert_q1=begin
dataset file T_ZY_JG_PROCESS1_1_dataset
(
schema: T_ZY_JG_PROCESS_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_jg_process/insert/insert.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_JG_PROCESS_syncher
(
inputs: T_ZY_JG_PROCESS1_1_dataset,
database:db,
operator:add,
table:T_ZY_JG_PROCESS
);
end
t_gg_sj_goods_insert_q1=begin
dataset file T_GG_SJ_GOODS2_dataset
(
schema: "T_GG_SJ_GOODS_schema",
filename: "/home/natt/syncdata/oraclesyncdata/t_gg_sj_goods/insert/insert.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_GG_SJ_GOODS_syncher
(
inputs: T_GG_SJ_GOODS2_dataset,
database:db,
operation:add,
table:T_GG_SJ_GOODS,
stream:true,
services :
(
(dataprocname : T_GG_SJ_GOODS_dataset,
serviceid: idx_job)
)
);
end
t_zy_rk_inbilldetail_insert_q1=begin
dataset file T_ZY_RK_INBILLDETAIL2_dataset
(
schema: T_ZY_RK_INBILLDETAIL_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_rk_inbilldetail/insert/insert.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_RK_INBILLDETAIL_syncher
(
inputs: T_ZY_RK_INBILLDETAIL2_dataset,
database:db,
operation:add,
table:T_ZY_RK_INBILLDETAIL,
stream:true,
services :
(
(dataprocname : T_ZY_RK_INBILLDETAIL_dataset,
serviceid: idx_job)
)
);
end
t_zy_jg_procarticledtl_insert_q1=begin
dataset file T_ZY_JG_PROCARTICLEDTL2_dataset
(
schema: T_ZY_JG_PROCARTICLEDTL_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_jg_procarticledtl/insert/insert.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_JG_PROCARTICLEDTL_syncher
(
inputs: T_ZY_JG_PROCARTICLEDTL2_dataset,
database:db,
operation:add,
table:T_ZY_JG_PROCARTICLEDTL,
stream:true,
services :
(
(dataprocname : T_ZY_JG_PROCARTICLEDTL_dataset,
serviceid: idx_job)
)
);
end
t_zy_gh_changeownerlist_insert_q1=begin
dataset file T_ZY_GH_CHANGEOWNERLIST2_dataset
(
schema: T_ZY_GH_CHANGEOWNERLIST_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_gh_changeownerlist/insert/insert.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_GH_CHANGEOWNERLIST_syncher
(
inputs: T_ZY_GH_CHANGEOWNERLIST2_dataset,
database:db,
operation:add,
table:T_ZY_GH_CHANGEOWNERLIST,
stream:true,
services :
(
(dataprocname : T_ZY_GH_CHANGEOWNERLIST_dataset,
serviceid: idx_job)
)
);
end
t_zy_jg_procmaterialdtl_insert_q1=begin
dataset file T_ZY_JG_PROCMATERIALDTL2_dataset
(
schema: T_ZY_JG_PROCMATERIALDTL_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_jg_procmaterialdtl/insert/insert.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_JG_PROCMATERIALDTL_syncher
(
inputs: T_ZY_JG_PROCMATERIALDTL2_dataset,
database:db,
operation:add,
table:T_ZY_JG_PROCMATERIALDTL,
stream:true,
services :
(
(dataprocname : T_ZY_JG_PROCMATERIALDTL_dataset,
serviceid: idx_job)
)
);
end
t_zy_gh_changeownerdetail_insert_q1=begin
dataset file T_ZY_GH_CHANGEOWNERDETAIL2_dataset
(
schema: T_ZY_GH_CHANGEOWNERDETAIL_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_gh_changeownerdetail/insert/insert.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_GH_CHANGEOWNERDETAIL_syncher
(
inputs: T_ZY_GH_CHANGEOWNERDETAIL2_dataset,
database:db,
operation:add,
table:T_ZY_GH_CHANGEOWNERDETAIL,
stream:true,
services :
(
(dataprocname : T_ZY_GH_CHANGEOWNERDETAIL_dataset,
serviceid: idx_job)
)
);
end
t_zy_ck_outpack_insert_q1=begin
dataset file T_ZY_CK_OUTPACK2_dataset
(
schema: T_ZY_CK_OUTPACK_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_ck_outpack/insert/insert.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_CK_OUTPACK_syncher
(
inputs: T_ZY_CK_OUTPACK2_dataset,
database:db,
operation:add,
table:T_ZY_CK_OUTPACK,
stream:true,
services :
(
(dataprocname : T_ZY_CK_OUTPACK_dataset,
serviceid: idx_job)
)
);
end
t_zy_ck_clear_delete_q1=begin
dataset file T_ZY_CK_CLEAR2_1_dataset
(
schema: "T_ZY_CK_CLEAR_schema",
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_ck_clear/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_CK_CLEAR_syncher
(
inputs: T_ZY_CK_CLEAR2_1_dataset,
database:db,
operator:delete,
table:T_ZY_CK_CLEAR
);
end
t_zy_jg_process_delete_q1=begin
dataset file T_ZY_JG_PROCESS2_1_dataset
(
schema: T_ZY_JG_PROCESS_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_jg_process/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_JG_PROCESS_syncher
(
inputs: T_ZY_JG_PROCESS2_1_dataset,
database:db,
operator:delete,
table:T_ZY_JG_PROCESS
);
end
t_gg_sj_goods_delete_q1=begin
dataset file T_GG_SJ_GOODS3_dataset
(
schema: "T_GG_SJ_GOODS_schema",
filename: "/home/natt/syncdata/oraclesyncdata/t_gg_sj_goods/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_GG_SJ_GOODS_syncher
(
inputs: T_GG_SJ_GOODS3_dataset,
database:db,
operation:delete,
table:T_GG_SJ_GOODS,
stream:true,
services :
(
(dataprocname : T_GG_SJ_GOODS_del_dataset,
serviceid: idx_job1)
)
);
end
t_zy_rk_inbilldetail_delete_q1=begin
dataset file T_ZY_RK_INBILLDETAIL3_dataset
(
schema: T_ZY_RK_INBILLDETAIL_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_rk_inbilldetail/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_RK_INBILLDETAIL_syncher
(
inputs: T_ZY_RK_INBILLDETAIL3_dataset,
database:db,
operation:delete,
table:T_ZY_RK_INBILLDETAIL,
stream:true,
services :
(
(dataprocname : T_ZY_RK_INBILLDETAIL_del_dataset,
serviceid: idx_job1)
)
);
end
t_zy_jg_procarticledtl_delete_q1=begin
dataset file T_ZY_JG_PROCARTICLEDTL3_dataset
(
schema: T_ZY_JG_PROCARTICLEDTL_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_jg_procarticledtl/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_JG_PROCARTICLEDTL_syncher
(
inputs: T_ZY_JG_PROCARTICLEDTL3_dataset,
database:db,
operation:delete,
table:T_ZY_JG_PROCARTICLEDTL,
stream:true,
services :
(
(dataprocname : T_ZY_JG_PROCARTICLEDTL_del_dataset,
serviceid: idx_job1)
)
);
end
t_zy_gh_changeownerlist_delete_q1=begin
dataset file T_ZY_GH_CHANGEOWNERLIST3_dataset
(
schema: T_ZY_GH_CHANGEOWNERLIST_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_gh_changeownerlist/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_GH_CHANGEOWNERLIST_syncher
(
inputs: T_ZY_GH_CHANGEOWNERLIST3_dataset,
database:db,
operation:delete,
table:T_ZY_GH_CHANGEOWNERLIST,
stream:true,
services :
(
(dataprocname : T_ZY_GH_CHANGEOWNERLIST_del_dataset,
serviceid: idx_job1)
)
);
end
t_zy_jg_procmaterialdtl_delete_q1=begin
dataset file T_ZY_JG_PROCMATERIALDTL3_dataset
(
schema: T_ZY_JG_PROCMATERIALDTL_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_jg_procmaterialdtl/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_JG_PROCMATERIALDTL_syncher
(
inputs: T_ZY_JG_PROCMATERIALDTL3_dataset,
database:db,
operation:delete,
table:T_ZY_JG_PROCMATERIALDTL,
stream:true,
services :
(
(dataprocname : T_ZY_JG_PROCMATERIALDTL_del_dataset,
serviceid: idx_job1)
)
);
end
t_zy_gh_changeownerdetail_delete_q1=begin
dataset file T_ZY_GH_CHANGEOWNERDETAIL3_dataset
(
schema: T_ZY_GH_CHANGEOWNERDETAIL_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_gh_changeownerdetail/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_GH_CHANGEOWNERDETAIL_syncher
(
inputs: T_ZY_GH_CHANGEOWNERDETAIL3_dataset,
database:db,
operation:delete,
table:T_ZY_GH_CHANGEOWNERDETAIL,
stream:true,
services :
(
(dataprocname : T_ZY_GH_CHANGEOWNERDETAIL_del_dataset,
serviceid: idx_job1)
)
);
end
t_zy_ck_outpack_delete_q1=begin
dataset file T_ZY_CK_OUTPACK3_dataset
(
schema: T_ZY_CK_OUTPACK_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_ck_outpack/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_CK_OUTPACK_syncher
(
inputs: T_ZY_CK_OUTPACK3_dataset,
database:db,
operation:delete,
table:T_ZY_CK_OUTPACK,
stream:true,
services :
(
(dataprocname : T_ZY_CK_OUTPACK_del_dataset,
serviceid: idx_job1)
)
);
end
<file_sep>/schema/T_OWNER.sql
CREATE SCHEMA T_OWNER_schema
SOURCE TYPE CSV
FIELDS(
"seg_no" type str,
"owner_num" type str,
"dept_id" type str,
"wprovider_id" type str,
"owner_id" type str,
"dept_subid" type str,
"use_flag" type str default value '0',
"wprovider_desc" type str,
"memberid" type str,
"wprovider_id_n" type str,
"deptid" type str,
"wprovider_address" type str,
"if_branch" type str
)
SCHEMA DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_OWNER_parser
TYPE rcd
SCHEMA T_OWNER_schema;
CREATE TABLE T_OWNER using T_OWNER_parser;
CREATE INDEX T_OWNER_index ON TABLE T_OWNER (seg_no);
<file_sep>/complete/JINSHANG-76/1_schema_jinshang76.sql
CREATE DATABASE db;
USE db;
create schema T_COMMON_DEPT_schema
source type CSV
fields(
"deptid" type str,
"deptcd" type str,
"deptname" type str,
"deptlevel" type double,
"deptdesc" type str,
"deptorder" type double,
"parentdeptid" type str,
"level1deptid" type str,
"level1deptcd" type str,
"level1deptname" type str,
"level2deptid" type str,
"level2deptcd" type str,
"level2deptname" type str,
"level3deptid" type str,
"level3deptcd" type str,
"level3deptname" type str,
"level4deptid" type str,
"level4deptcd" type str,
"level4deptname" type str,
"r_record_create_date" type DATETIME,
"r_record_create_user" type str,
"r_record_update_date" type DATETIME,
"r_record_update_user" type str,
"r_record_is_deleted" type double,
"r_record_change_flag" type double,
"deptaddress" type str,
"depttype" type str default value '22',
"districtid" type str,
"districtname" type str,
"belongarea" type str,
"firstdis" type str,
"opselected" type str default value '0',
"opupload" type str,
"limittime" type DATETIME,
"contractno" type str,
"baosaas_customer_name" type str
)
RECORD DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_COMMON_DEPT_parser
TYPE rcd
SCHEMA T_COMMON_DEPT_schema;
CREATE TABLE T_COMMON_DEPT using T_COMMON_DEPT_parser;
CREATE INDEX T_COMMON_DEPT_index ON TABLE T_COMMON_DEPT PRIMARY KEY(deptid);
CREATE MAP T_COMMON_DEPT_map ON table T_COMMON_DEPT
KEY (deptid)
VALUE (deptname)
TYPE string;
create schema T_BM_UI_CONFIGURE_schema
source type CSV
fields(
"manage_no" type str,
"deptid" type str,
"configure_no" type str,
"configure_desc" type str,
"configure_grade" type str,
"configure_node_no" type str,
"cf_value1" type str,
"cf_value2" type str,
"cf_value3" type double,
"cf_value4" type double,
"configure_remark" type str,
"r_record_create_date" type DATETIME,
"r_record_create_user" type str,
"r_record_update_date" type DATETIME,
"r_record_update_user" type str,
"r_record_is_deleted" type double,
"r_record_change_flag" type double,
"cf_value5" type DATETIME
)
RECORD DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_BM_UI_CONFIGURE_parser
TYPE rcd
SCHEMA T_BM_UI_CONFIGURE_schema;
CREATE TABLE T_BM_UI_CONFIGURE using T_BM_UI_CONFIGURE_parser;
CREATE INDEX T_BM_UI_CONFIGURE_index ON TABLE T_BM_UI_CONFIGURE PRIMARY KEY(manage_no);
CREATE MAP T_BM_UI_CONFIGURE_map ON table T_BM_UI_CONFIGURE
KEY (1)
VALUE (count(1))
TYPE u64
where configure_no = 'RPCONFIG' and deptid = '101';
create schema T_GG_SJ_GOODS_schema
source type CSV
fields(
"goodsid" type str,
"deptid" type str,
"packnum" type str,
"barcode" type str,
"goodslocid" type str,
"stocktype" type str,
"ownerid" type str,
"ownername" type str,
"goodsownermanageno" type str,
"inbillid" type str,
"goodsownerpackno" type str,
"manageno" type str,
"feebegindate" type DATETIME,
"contractid" type str,
"goodsstateno" type str,
"goodsstatename" type str,
"changeownertimes" type double default value '0',
"goodstype" type str,
"goodstypename" type str,
"kindno" type str,
"kindname" type str,
"shopsignid" type str,
"shopsignname" type str,
"prodareaid" type str,
"prodareaname" type str,
"goodsbatchno" type str,
"prodstoveno" type str,
"gradenum" type str,
"gradenumthick" type double,
"gradenumwidth" type double,
"gradenumlength" type double,
"grossweight" type double,
"netweight" type double,
"poundweight" type double,
"checkweight" type double,
"sheetnum" type double default value '1',
"unit" type str,
"packtype" type str,
"stockareaid" type str,
"qualitymemo" type str,
"firstinownerid" type str,
"firstindate" type DATETIME,
"outbillid" type str,
"ldglistid" type str,
"materialgoodsid" type str,
"intype" type str,
"outtype" type str,
"billstate" type double,
"outbilltime" type DATETIME,
"memo" type str,
"extsysplanobjectid" type str,
"extsyskindid" type str,
"extsysshopsignid" type str,
"extsysprodareaid" type str,
"foreignlistno" type str,
"sendflag" type double,
"r_record_create_date" type DATETIME,
"r_record_create_user" type str,
"r_record_update_date" type DATETIME,
"r_record_update_user" type str,
"r_record_is_deleted" type double,
"incusttime" type DATETIME,
"sidemark" type str,
"temp" type str,
"length_product_max" type double,
"heat_num" type str,
"test_lot_num" type str,
"r_record_change_flag" type double,
"sdprice" type str,
"extsysgradenum" type str,
"collectionmanname" type str,
"lockflag" type str,
"check_type" type str,
"outdate" type DATETIME,
"yfweight" type double,
"yfsheet" type double,
"pregoodsid" type str,
"issplit" type double,
"gradenumxh" type double,
"gradenumwhdw" type str,
"splittime" type double,
"isclear" type double,
"singlesheetnum" type double,
"gradenumhz" type str,
"gradenumthickhz" type double,
"singlesheet" type double,
"loclv1" type str,
"singlesheetunit" type str,
"dcemp" type str,
"qzemp" type str,
"dcemp2" type str,
"shipno" type str,
"shipname" type str,
"shipcd" type str,
"shiplevel" type str,
"cmmemo" type str,
"cmmemock" type str,
"shopsigncd" type str,
"processtypename" type str,
"packtypename" type str,
"sort_emp" type str,
"customerid" type str,
"lvgoodsid" type str,
"dispersesheet" type double,
"dbdeptid" type str,
"dbcustomer" type str,
"dbgulender" type str,
"locked_object" type str,
"locked_date" type DATETIME,
"unlocked_date" type DATETIME,
"preownername" type str,
"ply" type double default value '0',
"tdstate" type str default value '1',
"opselected" type str,
"oplock" type str,
"opinupload" type str,
"opchangeupload" type str,
"opinbatchid" type str,
"opchangebatchid" type str,
"opinupload_date" type DATETIME,
"out_package_status" type str,
"out_package_desc" type str,
"gmpacktype" type str,
"handno" type str,
"saveno" type str,
"drawno" type str,
"dangerouslevel" type str,
"acceptancecriteria" type str,
"shippingmark" type str,
"loclv2" type str,
"payedflag" type str,
"ordergoodsownerid" type str,
"ordergoodsownername" type str,
"vf_deptname" type `expr` data type string value iilmap("T_COMMON_DEPT_map",deptid),
"vf_count" type `expr` data type string value iilmap("T_BM_UI_CONFIGURE_map",1)
)
RECORD DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_GG_SJ_GOODS_parser
TYPE rcd
SCHEMA T_GG_SJ_GOODS_schema;
CREATE TABLE T_GG_SJ_GOODS using T_GG_SJ_GOODS_parser;
create schema T_GG_SJ_GOODS1_schema
source type CSV
fields(
"deptid" type str,
"vf_deptname" type str,
"gradenum" type str,
"shopsignid" type str,
"shopsignname" type str,
"prodareaid" type str,
"prodareaname" type str,
"kindno" type str,
"kindname" type str,
"ownername" type str,
"goodsownerpackno" type str,
"sumnetweight" type str,
"sumgrossweight" type str,
"sumsheetnum" type str,
"vf_count" type str,
"vf_countcase" type str
)
RECORD DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_GG_SJ_GOODS1_parser
TYPE rcd
SCHEMA T_GG_SJ_GOODS1_schema;
CREATE TABLE T_GG_SJ_GOODS1 using T_GG_SJ_GOODS1_parser;
CREATE INDEX T_GG_SJ_GOODS1_index ON TABLE T_GG_SJ_GOODS1 PRIMARY KEY(goodsownerpackno);
<file_sep>/complete/JINSHANG-88/jinshang-88.sql
SELECT sum(t.netweightsum), COUNT (*)
FROM t_zy_gh_changeownerlist t
WHERE R_RECORD_CREATE_DATE>to_date(to_char(sysdate,'yyyy-mm-dd'),'yyyy-mm-dd')
AND t.jyzxflag=1;
<file_sep>/complete/JINSHANG-102/del.sql
create job t_zy_ck_clear_delete_q1(zykie)
begin
dataset file T_ZY_CK_CLEAR2_1_dataset
(
schema: "T_ZY_CK_CLEAR_schema",
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_ck_clear/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_CK_CLEAR_syncher
(
inputs: T_ZY_CK_CLEAR2_1_dataset,
database:db,
operator:delete,
table:T_ZY_CK_CLEAR
);
end;
run job t_zy_ck_clear_delete_q1(threads:8);
create job t_zy_jg_process_delete_q1(zykie)
begin
dataset file T_ZY_JG_PROCESS2_1_dataset
(
schema: T_ZY_JG_PROCESS_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_jg_process/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_JG_PROCESS_syncher
(
inputs: T_ZY_JG_PROCESS2_1_dataset,
database:db,
operator:delete,
table:T_ZY_JG_PROCESS
);
end;
run job t_zy_jg_process_delete_q1(threads:8);
create job t_gg_sj_goods_delete_q1(zykie)
begin
dataset file T_GG_SJ_GOODS3_dataset
(
schema: "T_GG_SJ_GOODS_schema",
filename: "/home/natt/syncdata/oraclesyncdata/t_gg_sj_goods/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_GG_SJ_GOODS_syncher
(
inputs: T_GG_SJ_GOODS3_dataset,
database:db,
operation:delete,
table:T_GG_SJ_GOODS,
stream:true,
services :
(
(dataprocname : T_GG_SJ_GOODS_del_dataset,
serviceid: idx_job1)
)
);
end;
run job t_gg_sj_goods_delete_q1(threads:8);
create job t_zy_rk_inbilldetail_delete_q1(zykie)
begin
dataset file T_ZY_RK_INBILLDETAIL3_dataset
(
schema: T_ZY_RK_INBILLDETAIL_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_rk_inbilldetail/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_RK_INBILLDETAIL_syncher
(
inputs: T_ZY_RK_INBILLDETAIL3_dataset,
database:db,
operation:delete,
table:T_ZY_RK_INBILLDETAIL,
stream:true,
services :
(
(dataprocname : T_ZY_RK_INBILLDETAIL_del_dataset,
serviceid: idx_job1)
)
);
end;
run job t_zy_rk_inbilldetail_delete_q1(threads:8);
create job t_zy_jg_procarticledtl_delete_q1(zykie)
begin
dataset file T_ZY_JG_PROCARTICLEDTL3_dataset
(
schema: T_ZY_JG_PROCARTICLEDTL_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_jg_procarticledtl/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_JG_PROCARTICLEDTL_syncher
(
inputs: T_ZY_JG_PROCARTICLEDTL3_dataset,
database:db,
operation:delete,
table:T_ZY_JG_PROCARTICLEDTL,
stream:true,
services :
(
(dataprocname : T_ZY_JG_PROCARTICLEDTL_del_dataset,
serviceid: idx_job1)
)
);
end;
run job t_zy_jg_procarticledtl_delete_q1(threads:8);
create job t_zy_gh_changeownerlist_delete_q1(zykie)
begin
dataset file T_ZY_GH_CHANGEOWNERLIST3_dataset
(
schema: T_ZY_GH_CHANGEOWNERLIST_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_gh_changeownerlist/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_GH_CHANGEOWNERLIST_syncher
(
inputs: T_ZY_GH_CHANGEOWNERLIST3_dataset,
database:db,
operation:delete,
table:T_ZY_GH_CHANGEOWNERLIST,
stream:true,
services :
(
(dataprocname : T_ZY_GH_CHANGEOWNERLIST_del_dataset,
serviceid: idx_job1)
)
);
end;
run job t_zy_gh_changeownerlist_delete_q1(threads:8);
create job t_zy_jg_procmaterialdtl_delete_q1(zykie)
begin
dataset file T_ZY_JG_PROCMATERIALDTL3_dataset
(
schema: T_ZY_JG_PROCMATERIALDTL_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_jg_procmaterialdtl/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_JG_PROCMATERIALDTL_syncher
(
inputs: T_ZY_JG_PROCMATERIALDTL3_dataset,
database:db,
operation:delete,
table:T_ZY_JG_PROCMATERIALDTL,
stream:true,
services :
(
(dataprocname : T_ZY_JG_PROCMATERIALDTL_del_dataset,
serviceid: idx_job1)
)
);
end;
run job t_zy_jg_procmaterialdtl_delete_q1(threads:8);
create job t_zy_gh_changeownerdetail_delete_q1(zykie)
begin
dataset file T_ZY_GH_CHANGEOWNERDETAIL3_dataset
(
schema: T_ZY_GH_CHANGEOWNERDETAIL_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_gh_changeownerdetail/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_GH_CHANGEOWNERDETAIL_syncher
(
inputs: T_ZY_GH_CHANGEOWNERDETAIL3_dataset,
database:db,
operation:delete,
table:T_ZY_GH_CHANGEOWNERDETAIL,
stream:true,
services :
(
(dataprocname : T_ZY_GH_CHANGEOWNERDETAIL_del_dataset,
serviceid: idx_job1)
)
);
end;
run job t_zy_gh_changeownerdetail_delete_q1(threads:8);
create job t_zy_ck_outpack_delete_q1(zykie)
begin
dataset file T_ZY_CK_OUTPACK3_dataset
(
schema: T_ZY_CK_OUTPACK_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_ck_outpack/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000)
);
dataproc syncher T_ZY_CK_OUTPACK_syncher
(
inputs: T_ZY_CK_OUTPACK3_dataset,
database:db,
operation:delete,
table:T_ZY_CK_OUTPACK,
stream:true,
services :
(
(dataprocname : T_ZY_CK_OUTPACK_del_dataset,
serviceid: idx_job1)
)
);
end;
run job t_zy_ck_outpack_delete_q1(threads:8);
<file_sep>/uncomplete/JINSHANG-101/jinshang-101.sql
SELECT *
FROM
(SELECT ownerid,
'' ownername,
manageno,
packnum,
max(gradenum) gradenum,
max(goodslocid) goodslocid,
kindname,
shopsignname,
GOODSOWNERPACKNO,
prodareaname,
firstindate,
sum(gradenumxh) gradenumxh,
sum(singlesheetnum) singlesheetnum,
max(SideMark) SideMark,
max(ShipName) ShipName,
sum(netweight) netweight,
sum(grossweight) grossweight,
sum(sheetnum) sheetnum
FROM
( SELECT ownerid,
manageno,
packnum,
max(gradenum) gradenum,
max(goodslocid) goodslocid,
kindname,
shopsignname,
GOODSOWNERPACKNO,
prodareaname,
firstindate,
sum(gradenumxh) gradenumxh,
sum(singlesheetnum) singlesheetnum,
max(SideMark) SideMark,
max(ShipName) ShipName,
sum(netweight) netweight,
sum(grossweight) grossweight,
sum(sheetnum) sheetnum
FROM T_GG_SJ_Goods
WHERE goodsstateno='20'
AND r_record_is_deleted=0
AND deptid='0301'
GROUP BY ownerid,
manageno,
packnum,
kindname,
shopsignname,
GOODSOWNERPACKNO,
prodareaname,
firstindate
UNION ALL SELECT ownerid,manageno,packnum,max(gradenum) gradenum,max(goodslocid) goodslocid,kindname,shopsignname,GOODSOWNERPACKNO, prodareaname,firstindate,sum(gradenumxh) gradenumxh,sum(singlesheetnum) singlesheetnum,max(SideMark) SideMark,max(ShipName) ShipName, -sum(netweight) netweight,-sum(grossweight) grossweight,-sum(sheetnum) sheetnum
FROM T_ZY_RK_InBillDetail
WHERE deptid='0301'
AND goodsstateno='20'
AND firstindate>=to_date('2015/7/2','yyyy-mm-dd')
GROUP BY ownerid,
manageno,
packnum,
kindname,
shopsignname,
GOODSOWNERPACKNO,
prodareaname,
firstindate
UNION ALL SELECT ownerid,
manageno,
packnum,
max(gradenum) gradenum,
max(goodslocid) goodslocid,
kindname,
shopsignname,
GOODSOWNERPACKNO,
prodareaname,
firstindate,
0 gradenumxh,
0 singlesheetnum,NULL,NULL, -sum(netweight) netweight,-sum(grossweight) grossweight,-sum(sheetnum) sheetnum
FROM T_ZY_JG_ProcArticleDtl
WHERE deptid='0301'
AND firstindate>=to_date('2015/7/2','yyyy-mm-dd')
AND goodsstateno='130'
GROUP BY ownerid,
manageno,
packnum,
kindname,
shopsignname,
GOODSOWNERPACKNO,
prodareaname,
firstindate
UNION ALL SELECT newownerno aownerid,
managenum manageno,
packnum,
max(gradenum) gradenum,
max(goodslocid) goodslocid ,
kindname,
shopsignname,
GOODSOWNERPACKNUM,
prodareaname,
firstindate,
0 gradenumxh,
0 singlesheetnum,NULL,NULL, -sum(netweight) netweight,-sum(grossweight) grossweight,-sum(sheetnum) sheetnum
FROM
(SELECT a.newownerno,a.newownername,(CASE WHEN (managenum IS NULL
OR managenum = '') THEN premanagenum ELSE managenum END) managenum,
b.shopsignid,
b.shopsignname,
GOODSOWNERPACKNUM,
b.prodareaid,
b.prodareaname,
b.gradenum,
b.netweight,
b.grossweight,
b.sheetnum,
b.firstindate,
b.kindname,
b.goodslocid,
b.packnum,
b.goodsownermanagenum goodsownermanageno
FROM
(SELECT deptid,
ChangeOwnerID,
newownerno,
newownername
FROM T_ZY_GH_ChangeOwnerList
WHERE DeptID='0301'
AND changedate>=to_date('2015/7/2','yyyy-mm-dd')) a ,
T_ZY_GH_ChangeOwnerDetail b
WHERE a.changeownerid=b.changeownerno
AND a.deptid=b.deptid ) tb1
GROUP BY newownerno,
managenum,
packnum,
kindname,
shopsignname,
GOODSOWNERPACKNUM,
prodareaname,
firstindate
UNION ALL SELECT ownerno,
managenum manageno,
packnum,
max(gradenum) gradenum,
max(goodslocid) goodslocid,
kindname,
shopsignname,
GOODSOWNERPACKNUM,
prodareaname,
firstindate,
sum(gradenumxh) gradenumxh,
sum(singlesheetnum) singlesheetnum,
max(SideMark) SideMark,
max(ShipName) ShipName,
sum(netweight) netweight,
sum(grossweight) grossweight,
sum(sheetnum) sheetnum
FROM T_ZY_CK_OutPack
WHERE deptid='0301'
AND OutCustTime>=to_date('2015/7/2','yyyy-mm-dd')
GROUP BY ownerno,
managenum,
packnum,
kindname,
shopsignname,
GOODSOWNERPACKNUM,
prodareaname,
firstindate
UNION ALL SELECT ownerid,
manageno,
a.packnum,
max(gradenum) gradenum,
max(goodslocid) goodslocid,
kindname,
shopsignname,
GOODSOWNERPACKNO,
prodareaname,
firstindate,
sum(gradenumxh) gradenumxh,
sum(singlesheetnum) singlesheetnum,
max(SideMark) SideMark,
max(ShipName) ShipName,
sum(netweight) netweight,
sum(grossweight) grossweight,
sum(sheetnum) sheetnum
FROM T_GG_SJ_Goods a,
T_ZY_CK_CLEAR b
WHERE a.goodsid=b.goodsid
AND a.deptid='0301'
AND CREATDATE>=to_date('2015/7/2','yyyy-mm-dd')
GROUP BY ownerid,
manageno,
a.packnum,
kindname,
shopsignname,
GOODSOWNERPACKNO,
prodareaname,
firstindate
UNION ALL SELECT ownerid,
manageno,
packnum,
max(gradenum) gradenum,
max(goodslocid) goodslocid,
kindname,
shopsignname,
GOODSOWNERPACKNO,
prodareaname,
firstindate,
0 gradenumxh,
0 singlesheetnum,
NULL,
NULL,
sum(netweight) netweight,
sum(grossweight) grossweight,
sum(sheetnum) sheetnum
FROM T_ZY_JG_ProcMaterialDtl
WHERE deptid='0301'
AND EXISTS
(SELECT 1
FROM T_ZY_JG_PROCESS
WHERE ProcessID=T_ZY_JG_ProcMaterialDtl.ProcessID
AND deptid=T_ZY_JG_ProcMaterialDtl.deptid
AND OutCarBillTime>=to_date('2015/7/2','yyyy-mm-dd'))
GROUP BY ownerid,
manageno,
packnum,
kindname,
shopsignname,
GOODSOWNERPACKNO,
prodareaname,
firstindate
UNION ALL SELECT preownerno ownerid,
premanagenum manageno,
packnum,
max(gradenum) gradenum,
max(goodslocid) goodslocid,
kindname,
shopsignname,
GOODSOWNERPACKNUM,
prodareaname,
firstindate,
0 gradenumxh,
0 singlesheetnum,
NULL,
NULL,
sum(netweight) netweight,
sum(grossweight) grossweight,
sum(sheetnum) sheetnum
FROM
(SELECT a.preownerno,
a.proownername,
b.premanagenum,
b.netweight,
b.grossweight,
b.sheetnum,
b.shopsignid,
b.shopsignname,
b.prodareaid,
b.prodareaname,
GOODSOWNERPACKNUM,
b.gradenum,
b.firstindate,
b.kindname,
b.goodslocid,
b.packnum
FROM
(SELECT deptid,
ChangeOwnerID,
preownerno,
proownername
FROM T_ZY_GH_ChangeOwnerList
WHERE DeptID='0301'
AND changedate>=to_date('2015/7/2','yyyy-mm-dd')) a ,
T_ZY_GH_ChangeOwnerDetail b
WHERE a.changeownerid=b.changeownerno
AND a.deptid=b.deptid) tb1
GROUP BY preownerno,
premanagenum,
packnum,
kindname,
shopsignname,
GOODSOWNERPACKNUM,
prodareaname,
firstindate ) tbx
WHERE ownerid = 'KH00001'
AND netweight <> 0
GROUP BY ownerid,
manageno,
packnum,
kindname,
shopsignname,
GOODSOWNERPACKNO,
prodareaname,
firstindate)
WHERE netweight <> 0;
<file_sep>/uncomplete/JINSHANG-97/jinshang-97.sql
SELECT rptdate,
Sum(nt) nt,
Sum(injs) injs,
Sum(OUTWEIGHT) OUTWEIGHT,
Sum(outjs) outjs
FROM (SELECT To_char(FIRSTINDATE, 'yyyy-mm-dd') AS RPTDATE,
Sum(NETWEIGHT) AS NT,
Count(*) AS injs,
0 OUTWEIGHT,
0 outjs
FROM T_ZY_RK_InBillDetail
WHERE ( GOODSSTATENO = '20' )
AND ( DEPTID = '0301' )
AND ( FirstInDate >= To_date('2015/7/1', 'yyyy-mm-dd') )
AND ( FirstInDate < To_date('2015/9/1', 'yyyy-mm-dd') )
AND OWNERID = 'KH00001'
AND ( 1 = 2
OR MANAGENO LIKE 'M%' )
GROUP BY To_char(FIRSTINDATE, 'yyyy-mm-dd')
UNION ALL
SELECT To_char(b.CHANGEDATE, 'yyyy-mm-dd') AS rptdate,
Sum(NETWEIGHT) AS nt,
Count(*) AS injs,
0 OUTWEIGHT,
0 outjs
FROM T_ZY_GH_ChangeOwnerDetail a,
T_ZY_GH_ChangeOwnerlist b
WHERE a.DEPTID = b.deptid
AND a.CHANGEOWNERNO = b.CHANGEOWNERID
AND a.DeptID = '0301'
AND NEWOWNERNO = 'KH00001'
AND ( 1 = 2
OR a.PREMANAGENUM LIKE 'M%' )
AND changedate >= To_date('2015/7/1', 'yyyy-mm-dd')
AND changedate < To_date('2015/9/1', 'yyyy-mm-dd')
GROUP BY To_char(b.CHANGEDATE, 'yyyy-mm-dd')
UNION ALL
SELECT To_char(b.OUTCUSTTIME, 'yyyy-mm-dd') AS rptdate,
0 nt,
0 injs,
Sum(a.NETWEIGHT) AS OUTWEIGHT,
COUNT AS outjs
FROM t_zy_ck_outPack a,
t_zy_ck_outbill b
WHERE a.deptid = b.deptid
AND a.OUTBILLNO = b.OUTBILLID
AND a.DEPTID = '0301'
AND a.OWNERNO = 'KH00001'
AND ( 1 = 2
OR MANAGENUM LIKE 'M%' )
AND a.GOODSSTATENO = '40'
AND b.outcusttime >= To_date('2015/7/1', 'yyyy-mm-dd')
AND b.outcusttime < To_date('2015/9/1', 'yyyy-mm-dd')
GROUP BY To_char(b.outcusttime, 'yyyy-mm-dd')
UNION ALL
SELECT To_char(b.changedate, 'yyyy-mm-dd') AS rptdate,
0 nt,
0 injs,
Sum(netweight) AS OUTWEIGHT,
Count(*) AS outjs
FROM T_ZY_GH_ChangeOwnerDetail a,
T_ZY_GH_ChangeOwnerlist b
WHERE a.deptid = b.deptid
AND a.changeownerno = b.changeownerid
AND a.DeptID = '0301'
AND preownerno = 'KH00001'
AND ( 1 = 2
OR b.newmanagenum LIKE 'M%' )
AND changedate >= To_date('2015/7/1', 'yyyy-mm-dd')
AND changedate < To_date('2015/9/1', 'yyyy-mm-dd')
GROUP BY To_char(b.changedate, 'yyyy-mm-dd'))
GROUP BY rptdate;
<file_sep>/complete/JINSHANG-110/del_1.sql
create job del_1_job(2)
begin
dataset file T_GG_SJ_GOODS_dataset_22
(
schema: T_GG_SJ_GOODS_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_gg_sj_goods/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc select union_select
(
inputs: "T_GG_SJ_GOODS_dataset_22",
fields:
(
(fname:"netweight",alias:"store_amount",type:"double"),
(fname:"0",alias:"store_in_amount",type:"double"),
(fname:"0",alias:"store_out_amount",type:"double"),
(fname:"0",alias:"store_process_amount",type:"double")
),
distinct: true,
data_opr:delete,
cache:true,
conditions:" goodsstateno = '110'
AND deptid NOT LIKE '03%'
AND deptid NOT LIKE 'ZZ%'
AND deptid NOT LIKE 'MG%' "
);
dataproc statistics T_GG_SJ_GOODS_statistics_del
(
inputs:union_select,
table:union_all,
data_opr:delete
);
end;
run job del_1_job (threads:8);
<file_sep>/schema/T_GG_SJ_CUSTOMER.sql
create schema T_GG_SJ_CUSTOMER_schema
source type CSV
fields(
"ownerno" type str,
"deptid" type str,
"ownername" type str,
"ownernamecut" type str,
"ownershort" type str,
"ownertypeno" type str,
"sysno" type str,
"email" type str,
"tel" type str,
"linkman" type str,
"fax" type str,
"postalcode" type str,
"netaddress" type str,
"address" type str,
"bankname" type str,
"bankaccounts" type str,
"taxpayerno" type str,
"flag" type str,
"remark" type str,
"minstorage" type double,
"accountreceivable" type double,
"r_record_create_date" type DATETIME,
"r_record_create_user" type str,
"r_record_update_date" type DATETIME,
"r_record_update_user" type str,
"r_record_is_deleted" type double,
"r_record_change_flag" type double,
"memberid" type str,
"isbaosteel" type str,
"tellink" type double,
"invoicekindid" type str,
"invoicekindname" type str,
"jgfeeflag" type str,
"minweight" type double,
"ccfeeflag" type str,
"townerno" type str,
"saveimage" type str,
"bwlno" type str,
"username" type str,
"sendemail" type str
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "NULL";
CREATE PARSER T_GG_SJ_CUSTOMER_parser
TYPE rcd
SCHEMA T_GG_SJ_CUSTOMER_schema;
CREATE TABLE T_GG_SJ_CUSTOMER using T_GG_SJ_CUSTOMER_parser;
CREATE INDEX T_GG_SJ_CUSTOMER_index ON TABLE T_GG_SJ_CUSTOMER (ownerno);<file_sep>/schema/T_ZY_JG_PROCARTICLEDTL.sql
use db;
create schema T_ZY_JG_PROCARTICLEDTL_schema
source type CSV
fields(
"procarticledtlid" type str,
"deptid" type str,
"processid" type str,
"packnum" type str,
"barcode" type str,
"goodslocid" type str,
"ownerid" type str,
"ownername" type str,
"goodsownermanageno" type str,
"goodsownerpackno" type str,
"manageno" type str default value "0" ,
"feebegindate" type DATETIME,
"contractid" type str,
"goodsstateno" type str,
"goodsstatename" type str,
"changeownertimes" type double,
"goodstype" type str,
"goodstypename" type str,
"kindno" type str,
"kindname" type str,
"shopsignid" type str,
"shopsignname" type str,
"prodareaid" type str,
"prodareaname" type str,
"goodsbatchno" type str,
"prodstoveno" type str,
"gradenum" type str,
"gradenumthick" type double,
"gradenumwidth" type double,
"gradenumlength" type double,
"grossweight" type double,
"netweight" type double,
"poundweight" type double,
"checkweight" type double,
"sheetnum" type double,
"packtype" type str,
"packtypename" type str,
"unit" type str,
"qualitymemo" type str,
"firstinownerid" type str,
"firstindate" type DATETIME,
"printflag" type str,
"quality" type str,
"qamember" type str,
"qaflag" type str,
"processqualityid" type str,
"r_record_create_date" type DATETIME,
"r_record_create_user" type str,
"r_record_update_date" type DATETIME,
"r_record_update_user" type str,
"r_record_is_deleted" type double,
"orderby" type double,
"r_record_change_flag" type double,
"instruction_id" type str,
"sendflag" type double,
"acceptance_times" type double,
"acceptancer" type str,
"acceptance_time" type DATETIME,
"skinweight" type double,
"cyyweight" type double,
"goodsid" type str,
"articlememo1" type str,
"articlememo2" type str,
"articlememo3" type str,
"articlememo4" type str,
"articlememo5" type str,
"articlememo6" type str,
"articlememo7" type str,
"articlememo8" type str,
"articlememo9" type str,
"articlememo10" type str,
"articleys" type str,
"customerid" type str,
"cbxjgtype" type str,
"wallwidth" type double,
"woodtype" type str,
"woodtypename" type str,
"chanxian" type str,
"drawno" type str,
"zcflag" type str default value "0",
"classgroup" type str,
"loclv1" type str,
"loclv2" type str
)
RECORD DELIMITER "CRLF"
FIELD DELIMITER ","
TEXT QUALIFIER "NULL";
CREATE PARSER T_ZY_JG_PROCARTICLEDTL_parser
TYPE rcd
SCHEMA T_ZY_JG_PROCARTICLEDTL_schema;
CREATE TABLE T_ZY_JG_PROCARTICLEDTL using T_ZY_JG_PROCARTICLEDTL_parser;
CREATE INDEX T_ZY_JG_PROCARTICLEDTL_index ON TABLE T_ZY_JG_PROCARTICLEDTL(customerid); <file_sep>/processing/JINSHANG-75/jinshang-75.sql
SELECT *
FROM
(SELECT nvl(GOODSID, 'null') AS GOODSID,
nvl(DEPTID, 'null') AS DEPTID,
(SELECT deptname
FROM wmsdba.t_common_dept d
WHERE d.deptid = g.deptid) AS DEPTNAME,
nvl(BARCODE, 'null') AS BARCODE,
nvl(GOODSLOCID, 'null') AS GOODSLOCID,
nvl(decode(STOCKTYPE, '004001', '室内库', '004002', '室外库', NULL, '室内库'), 'null') AS STOCKTYPE,
nvl(OWNERID, 'null') AS OWNERID,
nvl(OWNERNAME, 'null') AS OWNERNAME,
nvl(GOODSOWNERMANAGENO, 'null') AS GOODSOWNERMANAGENO,
nvl(INBILLID, 'null') AS INBILLID,
nvl(PACKNUM, 'null') AS PACKNUM,
nvl(GOODSOWNERPACKNO, 'null') AS GOODSOWNERPACKNO,
nvl(MANAGENO, 'null') AS MANAGENO,
nvl(to_char(FEEBEGINDATE, 'YYYY-MM-DD hh24:mi:ss'), 'null') AS FEEBEGINDATE,
nvl(CONTRACTID, 'null') AS CONTRACTID,
nvl(GOODSSTATENO, 'null') AS GOODSSTATENO,
nvl(GOODSSTATENAME, 'null') AS GOODSSTATENAME,
nvl(to_char(CHANGEOWNERTIMES), 'null') AS CHANGEOWNERTIMES,
nvl(GOODSTYPE, 'null') AS GOODSTYPE,
nvl(GOODSTYPENAME, 'null') AS GOODSTYPENAME,
nvl(KINDNO, 'null') AS KINDNO,
nvl(KINDNAME, 'null') AS KINDNAME,
nvl(SHOPSIGNID, 'null') AS SHOPSIGNID,
nvl(SHOPSIGNNAME, 'null') AS SHOPSIGNNAME,
nvl(PRODAREAID, 'null') AS PRODAREAID,
nvl(PRODAREANAME, 'null') AS PRODAREANAME,
nvl(GOODSBATCHNO, 'null') AS GOODSBATCHNO,
nvl(PRODSTOVENO, 'null') AS PRODSTOVENO,
nvl(GRADENUM, 'null') AS GRADENUM,
nvl(GRADENUMTHICK, 0) AS GRADENUMTHICK,
nvl(to_char(GRADENUMWIDTH), 'null') AS GRADENUMWIDTH,
nvl(to_char(GRADENUMLENGTH), 'null') AS GRADENUMLENGTH,
nvl(GROSSWEIGHT, 0) AS GROSSWEIGHT,
nvl(NETWEIGHT, 0) AS NETWEIGHT,
nvl(POUNDWEIGHT, 0) AS POUNDWEIGHT,
nvl(CHECKWEIGHT, 0) AS CHECKWEIGHT,
nvl(SHEETNUM, 0) AS SHEETNUM,
nvl(UNIT, 'null') AS UNIT,
nvl(PACKTYPE, 'null') AS PACKTYPE,
nvl(STOCKAREAID, 'null') AS STOCKAREAID,
nvl(QUALITYMEMO, 'null') AS QUALITYMEMO,
nvl(FIRSTINOWNERID, 'null') AS FIRSTINOWNERID,
nvl(to_char(FIRSTINDATE, 'YYYY-MM-DD hh24:mi:ss'), 'null') AS FIRSTINDATE,
nvl(OUTBILLID, 'null') AS OUTBILLID,
nvl(LDGLISTID, 'null') AS LDGLISTID,
nvl(MATERIALGOODSID, 'null') AS MATERIALGOODSID,
nvl(decode(INTYPE, '20', '正常入库', '50', '退货入库', '99', '其它'), 'null') AS INTYPE,
nvl(OUTTYPE, 'null') AS OUTTYPE,
nvl(to_char(BILLSTATE), 'null') AS BILLSTATE,
nvl(to_char(OUTBILLTIME, 'YYYY-MM-DD hh24:mi:ss'), 'null') AS OUTBILLTIME,
nvl(MEMO, 'null') AS MEMO,
nvl(SDPRICE, 'null') AS SDPRICE,
nvl(SIDEMARK, 'null') AS TRUCKNO,
nvl(to_char(OUTBILLTIME, 'YYYY-MM-DD hh24:mi:ss'), 'null') AS OUTCUSTTIME,
nvl(to_char(round(sysdate - FIRSTINDATE)), 'null') AS STORAGEDAY,
nvl(to_char(LENGTH_PRODUCT_MAX), 'null') AS LENGTHMAX
FROM wmsdba.t_gg_sj_goods g
WHERE 1 = 1
AND GOODSID = 'ww'
AND PACKNUM = 'ww'
AND deptId = 'ww')
WHERE rownum <= 20<file_sep>/schema/T_WL_STORE.sql
CREATE SCHEMA T_WL_STORE_schema
SOURCE TYPE CSV
FIELDS(
"dept_id" type str,
"store_short_name" type str,
"lng" type double,
"lat" type double,
"hasvideo" type double default value "0",
"storelimit" type double
)
SCHEMA DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_WL_STORE_parser
TYPE rcd
SCHEMA T_WL_STORE_schema;
CREATE TABLE T_WL_STORE using T_WL_STORE_parser;
CREATE INDEX T_WL_STORE_index ON TABLE T_WL_STORE (dept_id);
<file_sep>/complete/JINSHANG-76/2_init_jinsahng76.sql
create job T_COMMON_DEPT_job(2)
begin
dataset file T_COMMON_DEPT_dataset
(
schema: "T_COMMON_DEPT_schema",
filename: "/home/natt/Data/jinshang_Data_New/T_COMMON_DEPT.csv",
serverid: "0",
splitter: (block_size: 10000000)
);
dataproc syncher T_COMMON_DEPT_syncher
(
inputs: T_COMMON_DEPT_dataset,
database:db,
operator:add,
table:T_COMMON_DEPT
);
end;
run job T_COMMON_DEPT_job(threads:8);
create job T_BM_UI_CONFIGURE_job(2)
begin
dataset file T_BM_UI_CONFIGURE_dataset
(
schema: "T_BM_UI_CONFIGURE_schema",
filename: "/home/natt/Data/jinshang_Data_New/T_BM_UI_CONFIGURE.csv",
serverid: "0",
splitter: (block_size: 10000000)
);
dataproc syncher T_BM_UI_CONFIGURE2_syncher
(
inputs: T_BM_UI_CONFIGURE_dataset,
database:db,
operator:add,
table:T_BM_UI_CONFIGURE
);
end;
run job T_BM_UI_CONFIGURE_job(threads:8);
create job T_GG_SJ_GOODS_job(2)
begin
dataset file T_GG_SJ_GOODS_dataset
(
schema:T_GG_SJ_GOODS_schema,
filename:"/home/natt/Data/jinshang_Data_New/T_GG_SJ_GOODS.csv",
serverid:0,
splitter:(block_size: 10000000)
);
dataproc select T_GG_SJ_GOODS_select
(
fields:
(
(fname:"nvl (deptid,'NULL')",alias:"deptid",type:"str"),
(fname:"iilmap('T_COMMON_DEPT_map')",alias:"vf_deptname",type:"str"),
(fname:"nvl (gradenum, 'NULL')",alias:"gradenum",type:"str"),
(fname:"nvl (shopsignid, 'NULL')",alias:"shopsignid",type:"str"),
(fname:"nvl (shopsignname, 'NULL')",alias:"shopsignname",type:"str"),
(fname:"nvl (prodareaid, 'NULL')",alias:"prodareaid",type:"str"),
(fname:"nvl (prodareaname, 'NULL')",alias:"prodareaname",type:"str"),
(fname:"nvl (kindno, 'NULL')",alias:"kindno",type:"str"),
(fname:"nvl (kindname, 'NULL')",alias:"kindname",type:"str"),
(fname:"nvl (ownername, 'NULL')",alias:"ownername",type:"str"),
(fname:"nvl (goodsownerpackno, 'NULL')",alias:"goodsownerpackno",type:"str"),
(fname:"nvl (netweight, 0)",alias:"netweight",type:"str"),
(fname:"nvl (grossweight, 0)",alias:"grossweight",type:"str"),
(fname:"nvl (sheetnum, 0)",alias:"sheetnum",type:"str"),
(fname:"iilmap('T_BM_UI_CONFIGURE_map',1)",alias:"vf_count",type:"str")
),
inputs: "T_GG_SJ_GOODS_dataset"
);
dataproc select T_GG_SJ_GOODS1_select
(
fields:
(
(fname:"deptid",type:"str"),
(fname:"vf_deptname",type:"str"),
(fname:"gradenum",type:"str"),
(fname:"shopsignid",type:"str"),
(fname:"shopsignname",type:"str"),
(fname:"prodareaid",type:"str"),
(fname:"prodareaname",type:"str"),
(fname:"kindno",type:"str"),
(fname:"kindname",type:"str"),
(fname:"ownername",type:"str"),
(fname:"goodsownerpackno",type:"str"),
(fname:"sum(netweight)",alias:"sumnetweight",type:"str"),
(fname:"sum(grossweight)",alias:"sumgrossweight",type:"str"),
(fname:"sum(sheetnum)",alias:"sumsheetnum",type:"str"),
(fname:"vf_count",type:"str"),
(fname:"case vf_count when '0' then '101%' else '101' end",alias:"vf_countcase",type:"str")
),
inputs: "T_GG_SJ_GOODS_select",
group_by:(deptid,vf_deptname,gradenum,shopsignid,shopsignname,prodareaid,prodareaname,kindno,kindname,ownername,goodsownerpackno,vf_count,vf_countcase)
);
dataproc syncher T_GG_SJ_GOODS1_syncher
(
inputs: T_GG_SJ_GOODS1_select,
database:db,
operator:add,
table:T_GG_SJ_GOODS1
);
end;
run job T_GG_SJ_GOODS_job (threads:8);
<file_sep>/uncomplete/JINSHANG-91/jinshang-91.sql
SELECT to_char(b.bill_date,'yyyy-mm-dd') A,
b.resource_num B,
b.bill_num C,
sum(b.unit_weight) D,
sum(c.netweight) E,
count(b.pack_num) F,
count(c.packnum) G,
b.out_settle_type H
FROM t_gg_tx_dl_bil_d b
LEFT JOIN v_t_gg_sj_goods_out c ON (c.packnum=b.pack_num
AND c.deptid=b.deptid)
WHERE b.deptid LIKE '03%'
AND b.owner_num LIKE '%GM0%'
AND to_char(b.bill_date,'yyyy-mm-dd')>='2015-07-01'
AND to_char(b.bill_date,'yyyy-mm-dd')<'2015-08-01'
GROUP BY to_char(b.bill_date,'yyyy-mm-dd'),
b.resource_num,
b.bill_num,
b.out_settle_type;
<file_sep>/processing/JINSHANG-76/del_3.sql
create job del_1_job(2)
begin
dataset file t_gg_sj_goods_dataset_22
(
schema: t_gg_sj_goods_schema,
filename: "/home/zhaoshun/Data/jingshang_data/T_GG_SJ_GOODS_del.txt",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc select t_gg_sj_goods_select
(
fields:
(
(fname:"GOODSID",type:"string"),
(fname:"DEPTID",type:"string"),
(fname:"GRADENUM",type:"string"),
(fname:"SHOPSIGNID",type:"string"),
(fname:"SHOPSIGNNAME",type:"string"),
(fname:"PRODAREAID",type:"string"),
(fname:"PRODAREANAME",type:"string"),
(fname:"KINDNO",type:"string"),
(fname:"KINDNAME",type:"string"),
(fname:"OWNERNAME",type:"string"),
(fname:"GOODSOWNERPACKNO",type:"string"),
(fname:"NETWEIGHT",type:"double"),
(fname:"GROSSWEIGHT",type:"double"),
(fname:"SHEETNUM",type:"double")
),
inputs: "t_gg_sj_goods_dataset_22",
distinct: true,
data_opr:delete,
cache:true
);
dataproc statistics T_GG_SJ_GOODS_statistics_del
(
inputs:t_gg_sj_goods_select,
table:t_gg_sj_goods,
data_opr:delete
);
end;
run job del_1_job (threads:8);
<file_sep>/schema/T_ZY_JS_SETTLEMENT.sql
create schema T_ZY_JS_SETTLEMENT_schema
SOURCE TYPE CSV
FIELDS(
"manageno" type str,
"settlecd" type str,
"deptid" type str,
"invoicekindid" type str,
"invoicekindname" type str,
"deptno" type str,
"deptname" type str,
"settledate" type datetime,
"invoicetypeid" type str,
"invoicetypename" type str,
"invoiceno" type str,
"chequeno" type str,
"chequesum" type double,
"chequeunit" type str,
"creditno" type str,
"creditamount" type double,
"cash" type double,
"earnest" type double,
"factsum" type double,
"borrow" type double,
"remit" type double,
"editor" type str,
"editorname" type str,
"creatdate" type datetime,
"flag" type double,
"memo" type str,
"resourceno" type str,
"invoiceflag" type double,
"r_record_create_date" type datetime,
"r_record_create_user" type str,
"r_record_update_date" type datetime,
"r_record_update_user" type str,
"r_record_is_deleted" type double,
"r_record_change_flag" type double,
"biz_id" type str,
"biz_type_id" type str,
"biz_type_name" type str,
"custom_id" type str,
"custom_name" type str,
"settle_data" type str,
"settle_weight" type str,
"should_sum" type double,
"sub_biz_type_id" type str,
"sub_biz_type_name" type str,
"weightsum" type double,
"grossweightsum" type double,
"ispay" type double,
"billempname" type str,
"foreignlistno" type str,
"jgbusinessno" type str,
"settledeptno" type str,
"settledeptname" type str,
"feekk" type double,
"kpusername" type str,
"kpamount" type double,
"kpdate" type datetime,
"settlelock" type str,
"jyzxflag" type double,
"jyzxsendflag" type double,
"jyzxfpsendflag" type double,
"jyzxispayfee" type double,
"sendmessage" type str,
"invoicebillno" type str,
"direction" type str,
"settletaxsum" type double,
"settlenotaxsum" type double
)
SCHEMA DELIMITER"LF"
FIELD DELIMITER","
TEXT QUALIFIER"DQM";
CREATE PARSER T_ZY_JS_SETTLEMENT_parser
TYPE rcd
SCHEMA T_ZY_JS_SETTLEMENT_schema;
CREATE TABLE T_ZY_JS_SETTLEMENT using T_ZY_JS_SETTLEMENT_parser;
create index T_ZY_JS_SETTLEMENT_index on table T_ZY_JS_SETTLEMENT(manageno);<file_sep>/schema/T_S_BILL_D.sql
CREATE SCHEMA T_S_BILL_D_schema
SOURCE TYPE CSV
FIELDS(
"seg_no" type str,
"bill_id" type str,
"bill_subid" type str,
"contract_id" type str,
"contract_subid" type str,
"s_resource_id" type str,
"good_seg_no" type str,
"good_id" type str,
"product_id" type str,
"factory_product_id" type str,
"plan_weight" type double,
"plan_qty" type double,
"weight_unit" type str,
"quantity_unit" type str,
"unit_conversion" type double,
"act_weight" type double,
"act_qty" type double,
"settle_weight" type double,
"settle_qty" type double,
"sale_price_notax" type double,
"settle_price_notax" type double,
"tax_rate" type double,
"freight_price" type double,
"out_price" type double,
"other_amount" type double,
"trans_charge_code" type str,
"store_charge_code" type str,
"ws_flag" type str default value '0',
"ws_date" type datetime,
"pack_id" type str,
"proc_mark" type str,
"insert_time" type datetime,
"spec" type str,
"wprovider_id" type str,
"downloadflag" type str default value '0',
"optflag" type str default value '0'
)
SCHEMA DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_S_BILL_D_parser
TYPE rcd
SCHEMA T_S_BILL_D_schema;
CREATE TABLE T_S_BILL_D using T_S_BILL_D_parser;
CREATE INDEX T_S_BILL_D_index ON TABLE T_S_BILL_D (seg_no);
<file_sep>/uncomplete/JINSHANG-104&106/jinshang-106.sql
SELECT kindname,
round(avg(sysdate-firstindate),0) avgday
FROM t_gg_sj_goods t
WHERE goodsstateno=20
AND EXISTS
(SELECT 1
FROM WL.T_OWNER W
WHERE SEG_NO='00103'
AND T.DEPTID=W.DEPTID
AND t.ownerid=w.owner_id
AND deptid NOT LIKE 'A%'
AND deptid!='0301'
AND deptid!='4501')
GROUP BY kindname;<file_sep>/complete/JINSHANG-110/add_2.sql
create job add_2_job(2)
begin
dataset file T_ZY_RK_INBILL_dataset_11
(
schema: T_ZY_RK_INBILL_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_zy_rk_inbill/insert/insert.csv",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc select union_select
(
inputs: "T_ZY_RK_INBILL_dataset_11",
fields:
(
(fname:"0",alias:"store_amount",type:"double"),
(fname:"netweightsum",alias:"store_in_amount",type:"double"),
(fname:"0",alias:"store_out_amount",type:"double"),
(fname:"0",alias:"store_process_amount",type:"double")
),
distinct: true,
cache:true,
conditions:" deptid NOT LIKE '03%'
AND deptid NOT LIKE 'ZZ%'
AND deptid NOT LIKE 'MG%' "
);
dataproc statistics T_ZY_RK_INBILL_add_statistics
(
inputs:union_select,
table:union_all
);
end;
run job add_2_job (threads:8);<file_sep>/complete/JINSHANG-107&108/jinshang-108.sql
SELECT sumdate,
sum(sumweight)
FROM t_chart_detailstorage
WHERE owner_num='PD'
AND sumdate>=to_char(sysdate-365,'yyyymmdd')
AND sumdate LIKE '______15'
AND deptid NOT LIKE 'A%'
AND deptid!='0301'
AND deptid!='4501'
GROUP BY sumdate
ORDER BY sumdate;<file_sep>/complete/JINSHANG-75/2_init.sql
create job t_common_dept_job(t_common)
begin
dataset file t_common_dept_dataset
(
schema:t_common_dept_schema,
filename: "/home/natt/Data/jinshang_Data_New/T_COMMON_DEPT.csv",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc syncher t_common_dept_syncher
(
inputs:t_common_dept_dataset,
database:db,
operator:add,
table:t_common_dept
);
end;
run job t_common_dept_job(threads:8);
create job t_gg_sj_goods_job(t_gg_sj)
begin
dataset file t_gg_sj_goods_dataset
(
schema:t_gg_sj_goods_schema,
filename:"/home/natt/Data/jinshang_Data_New/T_GG_SJ_GOODS.csv",
serverid: 0,
splitter:(block_size: 10000000)
);
dataproc syncher t_gg_sj_goods_syncher
(
inputs:t_gg_sj_goods_dataset,
database:db,
operator:add,
table:t_gg_sj_goods
);
end;
run job t_gg_sj_goods_job (threads:8);
<file_sep>/complete/JINSHANG-75/zidonghua.sql
t_common_dept_insert_q1=begin
dataset file t_common_dept1_dataset
(
schema:t_common_dept_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_common_dept/insert/insert.csv",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc syncher t_common_dept_syncher
(
inputs:t_common_dept1_dataset,
database:db,
operator:add,
table:t_common_dept
);
end
t_common_dept_delete_q1=begin
dataset file t_common_dept2_dataset
(
schema:t_common_dept_schema,
filename: "/home/natt/syncdata/oraclesyncdata/t_common_dept/delete/delete.csv",
serverid: 0,
splitter: (block_size: 10000000)
);
dataproc syncher t_common_dept_syncher
(
inputs:t_common_dept2_dataset,
database:db,
operator:delete,
table:t_common_dept
);
end
t_gg_sj_goods_insert_q1=begin
dataset file t_gg_sj_goods1_dataset
(
schema:t_gg_sj_goods_schema,
filename:"/home/natt/syncdata/oraclesyncdata/t_gg_sj_goods/insert/insert.csv",
serverid: 0,
splitter:(block_size: 10000000)
);
dataproc syncher t_gg_sj_goods_syncher
(
inputs:t_gg_sj_goods1_dataset,
database:db,
operator:add,
table:t_gg_sj_goods
);
end
t_gg_sj_goods_delete_q1=begin
dataset file t_gg_sj_goods2_dataset
(
schema:t_gg_sj_goods_schema,
filename:"/home/natt/syncdata/oraclesyncdata/t_gg_sj_goods/delete/delete.csv",
serverid: 0,
splitter:(block_size: 10000000)
);
dataproc syncher t_gg_sj_goods_syncher
(
inputs:t_gg_sj_goods2_dataset,
database:db,
operator:delete,
table:t_gg_sj_goods
);
end<file_sep>/uncomplete/JINSHANG-93/T_SI_LOGISTICS_READY_M.sql
CREATE SCHEMA T_SI_LOGISTICS_READY_M_schema
SOURCE TYPE CSV
FIELDS(
"seg_no" type str,
"ready_num" type str,
"order_num" type str,
"ready_date_chr" type str,
"ready_t" type str,
"weight_ready_tot" type double,
"n_ready_tot" type double,
"trnp_mode_code" type str,
"trnp_mode_name" type str,
"dlv_spot_code" type str,
"dlv_spot_name" type str,
"private_route_code" type str,
"private_route_name" type str,
"proc_mark" type str,
"time_receive" type datetime,
"provider_id" type str,
"provider_name" type str
)
SCHEMA DELIMITER "LF"
FIELD DELIMITER ","
TEXT QUALIFIER "DQM";
CREATE PARSER T_SI_LOGISTICS_READY_M_parser
TYPE rcd
SCHEMA T_SI_LOGISTICS_READY_M_schema;
CREATE TABLE T_SI_LOGISTICS_READY_M using T_SI_LOGISTICS_READY_M_parser;
CREATE INDEX T_SI_LOGISTICS_READY_M_index ON TABLE T_SI_LOGISTICS_READY_M (seg_no);
| 0647393cd7dfdd1aab5e79f2a013ad8a6dfef248 | [
"SQL"
] | 96 | SQL | Natt7/JINSHANG | f1108a27c68ca5d9590628213b058a5d8bc18b40 | 7800a524dfbcf42b74c6ce78f533909ae88d7003 | |
refs/heads/master | <repo_name>AlexJ-94/loan_calculator<file_sep>/loan_calculator.py
import json
def main():
names = json.loads(open('loan_information.json').read())
for name in names:
print(name)
main()
| 3a822793ac2aee38064da253d9b8e05ab5f767bf | [
"Python"
] | 1 | Python | AlexJ-94/loan_calculator | b38825fd08bf7db6efe0efcf3aed177f86c5fb78 | b377360f8f5501f248baef7d8209218d89da30e2 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.